diff --git a/openbb_terminal/common/behavioural_analysis/twitter_model.py b/openbb_terminal/common/behavioural_analysis/twitter_model.py
deleted file mode 100644
index 034cc8341131..000000000000
--- a/openbb_terminal/common/behavioural_analysis/twitter_model.py
+++ /dev/null
@@ -1,212 +0,0 @@
-"""Twitter Model"""
-__docformat__ = "numpy"
-
-import logging
-from datetime import datetime, timedelta
-from typing import Optional
-
-import pandas as pd
-from vaderSentiment.vaderSentiment import SentimentIntensityAnalyzer
-
-from openbb_terminal.core.session.current_user import get_current_user
-from openbb_terminal.decorators import check_api_key, log_start_end
-from openbb_terminal.helper_funcs import clean_tweet, get_data, request
-from openbb_terminal.rich_config import console
-
-logger = logging.getLogger(__name__)
-
-analyzer = SentimentIntensityAnalyzer()
-
-
-@log_start_end(log=logger)
-@check_api_key(["API_TWITTER_BEARER_TOKEN"])
-def load_analyze_tweets(
- symbol: str,
- limit: int = 100,
- start_date: Optional[str] = "",
- end_date: Optional[str] = "",
-) -> pd.DataFrame:
- """Load tweets from twitter API and analyzes using VADER.
-
- Parameters
- ----------
- symbol: str
- Ticker symbol to search twitter for
- limit: int
- Number of tweets to analyze
- start_date: Optional[str]
- If given, the start time to get tweets from
- end_date: Optional[str]
- If given, the end time to get tweets from
-
- Returns
- -------
- df_tweet: pd.DataFrame
- Dataframe of tweets and sentiment
- """
- params = {
- "query": rf"(\${symbol}) (lang:en)",
- "max_results": str(limit),
- "tweet.fields": "created_at,lang",
- }
-
- if start_date:
- # Assign from and to datetime parameters for the API
- params["start_time"] = start_date
- if end_date:
- params["end_time"] = end_date
-
- # Request Twitter API
- response = request(
- "https://api.twitter.com/2/tweets/search/recent",
- params=params, # type: ignore
- headers={
- "authorization": "Bearer "
- + get_current_user().credentials.API_TWITTER_BEARER_TOKEN
- },
- )
-
- # Create dataframe
- df_tweets = pd.DataFrame()
-
- # Check that the API response was successful
- if response.status_code == 200:
- tweets = []
- for tweet in response.json()["data"]:
- row = get_data(tweet)
- tweets.append(row)
- df_tweets = pd.DataFrame(tweets)
- elif response.status_code == 401:
- console.print("Twitter API Key provided is incorrect\n")
- return pd.DataFrame()
- elif response.status_code == 400:
- console.print(
- """
- Status Code 400.
- This means you are requesting data from beyond the API's 7 day limit"""
- )
- return pd.DataFrame()
- elif response.status_code == 403:
- console.print(
- f"""
- Status code 403.
- It seems you're twitter credentials are invalid - {response.text}
- """
- )
- return pd.DataFrame()
- else:
- console.print(
- f"""
- Status code {response.status_code}.
- Something went wrong - {response.text}
- """
- )
- return pd.DataFrame()
-
- sentiments = []
- pos = []
- neg = []
- neu = []
-
- for s_tweet in df_tweets["text"].to_list():
- tweet = clean_tweet(s_tweet, symbol)
- sentiments.append(analyzer.polarity_scores(tweet)["compound"])
- pos.append(analyzer.polarity_scores(tweet)["pos"])
- neg.append(analyzer.polarity_scores(tweet)["neg"])
- neu.append(analyzer.polarity_scores(tweet)["neu"])
- # Add sentiments to tweets dataframe
- df_tweets["sentiment"] = sentiments
- df_tweets["positive"] = pos
- df_tweets["negative"] = neg
- df_tweets["neutral"] = neu
-
- return df_tweets
-
-
-@log_start_end(log=logger)
-def get_sentiment(
- symbol: str,
- n_tweets: int = 15,
- n_days_past: int = 2,
-) -> pd.DataFrame:
- """Get sentiments from symbol.
-
- Parameters
- ----------
- symbol: str
- Stock ticker symbol to get sentiment for
- n_tweets: int
- Number of tweets to get per hour
- n_days_past: int
- Number of days to extract tweets for
-
- Returns
- -------
- df_sentiment: pd.DataFrame
- Dataframe of sentiment
- """
- # Date format string required by twitter
- dt_format = "%Y-%m-%dT%H:%M:%SZ"
-
- # Algorithm to extract
- dt_recent = datetime.utcnow() - timedelta(seconds=20)
- dt_old = dt_recent - timedelta(days=n_days_past)
- console.print(
- f"From {dt_recent.date()} retrieving {n_tweets*24} tweets ({n_tweets} tweets/hour)"
- )
-
- df_tweets = pd.DataFrame(
- columns=[
- "created_at",
- "text",
- "sentiment",
- "positive",
- "negative",
- "neutral",
- ]
- )
- while True:
- # Iterate until we haven't passed the old number of days
- if dt_recent < dt_old:
- break
- # Update past datetime
- dt_past = dt_recent - timedelta(minutes=60)
-
- temp = load_analyze_tweets(
- symbol,
- n_tweets,
- start_date=dt_past.strftime(dt_format),
- end_date=dt_recent.strftime(dt_format),
- )
-
- if (isinstance(temp, pd.DataFrame) and temp.empty) or (
- not isinstance(temp, pd.DataFrame) and not temp
- ):
- return pd.DataFrame()
-
- df_tweets = pd.concat([df_tweets, temp])
-
- if dt_past.day < dt_recent.day:
- console.print(
- f"From {dt_past.date()} retrieving {n_tweets*24} tweets ({n_tweets} tweets/hour)"
- )
-
- # Update recent datetime
- dt_recent = dt_past
-
- # Sort tweets per date
- df_tweets.sort_index(ascending=False, inplace=True)
- df_tweets["cumulative_compound"] = df_tweets["sentiment"].cumsum()
- df_tweets["prob_sen"] = 1
-
- # df_tweets.to_csv(r'notebooks/tweets.csv', index=False)
- df_tweets.reset_index(inplace=True)
- df_tweets["Month"] = pd.to_datetime(df_tweets["created_at"]).apply(
- lambda x: x.month
- )
- df_tweets["Day"] = pd.to_datetime(df_tweets["created_at"]).apply(lambda x: x.day)
- df_tweets["date"] = pd.to_datetime(df_tweets["created_at"])
- df_tweets = df_tweets.sort_values(by="date")
- df_tweets["cumulative_compound"] = df_tweets["sentiment"].cumsum()
-
- return df_tweets
diff --git a/openbb_terminal/common/behavioural_analysis/twitter_view.py b/openbb_terminal/common/behavioural_analysis/twitter_view.py
deleted file mode 100644
index fba727706288..000000000000
--- a/openbb_terminal/common/behavioural_analysis/twitter_view.py
+++ /dev/null
@@ -1,197 +0,0 @@
-"""Twitter view."""
-__docformat__ = "numpy"
-
-import logging
-import os
-from typing import Optional, Union
-
-import numpy as np
-import pandas as pd
-from dateutil import parser as dparse
-
-from openbb_terminal import OpenBBFigure, theme
-from openbb_terminal.common.behavioural_analysis import twitter_model
-from openbb_terminal.decorators import log_start_end
-from openbb_terminal.helper_funcs import export_data, get_closing_price
-from openbb_terminal.rich_config import console
-
-logger = logging.getLogger(__name__)
-
-
-@log_start_end(log=logger)
-def display_inference(
- symbol: str, limit: int = 100, export: str = "", sheet_name: Optional[str] = None
-):
- """Prints Inference sentiment from past n tweets.
-
- Parameters
- ----------
- symbol: str
- Stock ticker symbol
- limit: int
- Number of tweets to analyze
- sheet_name: str
- Optionally specify the name of the sheet the data is exported to.
- export: str
- Format to export tweet dataframe
- """
- df_tweets = twitter_model.load_analyze_tweets(symbol, limit)
-
- if (isinstance(df_tweets, pd.DataFrame) and df_tweets.empty) or (
- not isinstance(df_tweets, pd.DataFrame) and not df_tweets
- ):
- return
-
- # Parse tweets
- dt_from = dparse.parse(df_tweets["created_at"].values[-1])
- dt_to = dparse.parse(df_tweets["created_at"].values[0])
- console.print(f"From: {dt_from.strftime('%Y-%m-%d %H:%M:%S')}")
- console.print(f"To: {dt_to.strftime('%Y-%m-%d %H:%M:%S')}")
-
- console.print(f"{len(df_tweets)} tweets were analyzed.")
- dt_delta = dt_to - dt_from
- n_freq = dt_delta.total_seconds() / len(df_tweets)
- console.print(f"Frequency of approx 1 tweet every {round(n_freq)} seconds.")
-
- pos = df_tweets["positive"]
- neg = df_tweets["negative"]
-
- percent_pos = len(np.where(pos > neg)[0]) / len(df_tweets)
- percent_neg = len(np.where(pos < neg)[0]) / len(df_tweets)
- total_sent = np.round(np.sum(df_tweets["sentiment"]), 2)
- mean_sent = np.round(np.mean(df_tweets["sentiment"]), 2)
- console.print(f"The summed compound sentiment of {symbol} is: {total_sent}")
- console.print(f"The average compound sentiment of {symbol} is: {mean_sent}")
- console.print(
- f"Of the last {len(df_tweets)} tweets, {100*percent_pos:.2f} % had a higher positive sentiment"
- )
- console.print(
- f"Of the last {len(df_tweets)} tweets, {100*percent_neg:.2f} % had a higher negative sentiment"
- )
-
- export_data(
- export,
- os.path.dirname(os.path.abspath(__file__)),
- "infer",
- df_tweets,
- sheet_name,
- )
-
-
-@log_start_end(log=logger)
-def display_sentiment(
- symbol: str,
- n_tweets: int = 15,
- n_days_past: int = 2,
- compare: bool = False,
- export: str = "",
- sheet_name: Optional[str] = None,
- external_axes: bool = False,
-) -> Union[OpenBBFigure, None]:
- """Plots sentiments from symbol
-
- Parameters
- ----------
- symbol: str
- Stock ticker symbol to get sentiment for
- n_tweets: int
- Number of tweets to get per hour
- n_days_past: int
- Number of days to extract tweets for
- compare: bool
- Show corresponding change in stock price
- sheet_name: str
- Optionally specify the name of the sheet the data is exported to.
- export: str
- Format to export tweet dataframe
- external_axes: bool, optional
- Whether to return the figure object or not, by default False
- """
-
- df_tweets = twitter_model.get_sentiment(symbol, n_tweets, n_days_past)
-
- if df_tweets.empty:
- return None
-
- if compare:
- plots_kwargs = dict(
- rows=3,
- cols=1,
- shared_xaxes=True,
- vertical_spacing=0.05,
- row_heights=[0.2, 0.6, 0.2],
- )
- else:
- plots_kwargs = dict(
- rows=2,
- cols=1,
- shared_xaxes=True,
- vertical_spacing=0.05,
- row_heights=[0.6, 0.4],
- )
-
- fig = OpenBBFigure.create_subplots(**plots_kwargs) # type: ignore
-
- fig.add_scatter(
- x=pd.to_datetime(df_tweets["created_at"]),
- y=df_tweets["cumulative_compound"].values,
- row=1,
- col=1,
- )
-
- fig.set_yaxis_title("
Cumulative
VADER Sentiment", row=1, col=1)
-
- for _, day_df in df_tweets.groupby(by="Day"):
- day_df["time"] = pd.to_datetime(day_df["created_at"])
- day_df = day_df.sort_values(by="time")
- fig.add_scatter(
- x=day_df["time"],
- y=day_df["sentiment"].cumsum(),
- row=1,
- col=1,
- )
- fig.add_bar(
- x=df_tweets["date"],
- y=df_tweets["positive"],
- row=2,
- col=1,
- marker_color=theme.up_color,
- )
-
- fig.add_bar(
- x=df_tweets["date"],
- y=-1 * df_tweets["negative"],
- row=2,
- col=1,
- marker_color=theme.down_color,
- )
- fig.set_yaxis_title("VADER Polarity Scores", row=2, col=1)
-
- if compare:
- # get stock end price for each corresponding day if compare == True
- closing_price_df = get_closing_price(symbol, n_days_past)
- fig.add_scatter(
- x=closing_price_df["Date"],
- y=closing_price_df["Close"],
- name=pd.to_datetime(closing_price_df["Date"]).iloc[0].strftime("%Y-%m-%d"),
- row=3,
- col=1,
- )
- fig.set_yaxis_title("Stock Price", row=3, col=1)
-
- fig.update_layout(
- title=f"Twitter's {symbol} total compound sentiment over time is {round(np.sum(df_tweets['sentiment']), 2)}",
- xaxis=dict(type="date"),
- showlegend=False,
- )
-
- export_data(
- export,
- os.path.dirname(os.path.abspath(__file__)),
- "sentiment",
- df_tweets,
- sheet_name,
- fig,
- )
-
- return fig.show(external=external_axes)
diff --git a/openbb_terminal/core/sdk/controllers/stocks_sdk_controller.py b/openbb_terminal/core/sdk/controllers/stocks_sdk_controller.py
index 676362d2e6e3..4ddbb03f5a66 100644
--- a/openbb_terminal/core/sdk/controllers/stocks_sdk_controller.py
+++ b/openbb_terminal/core/sdk/controllers/stocks_sdk_controller.py
@@ -42,8 +42,6 @@ def ba(self):
`getdd`: Get due diligence posts from list of subreddits [Source: reddit].\n
`headlines`: Gets Sentiment analysis provided by FinBrain's API [Source: finbrain].\n
`headlines_chart`: Plots Sentiment analysis from FinBrain. Prints table if raw is True. [Source: FinBrain]\n
- `infer`: Load tweets from twitter API and analyzes using VADER.\n
- `infer_chart`: Prints Inference sentiment from past n tweets.\n
`mentions`: Get interest over time from google api [Source: google].\n
`mentions_chart`: Plots weekly bars of stock's interest over time. other users watchlist. [Source: Google].\n
`messages`: Get last messages for a given ticker [Source: stocktwits].\n
@@ -55,8 +53,6 @@ def ba(self):
`regions`: Get interest by region from google api [Source: google].\n
`regions_chart`: Plots bars of regions based on stock's interest. [Source: Google].\n
`rise`: Get top rising related queries with this stock's query [Source: google].\n
- `sentiment`: Get sentiments from symbol.\n
- `sentiment_chart`: Plots sentiments from symbol\n
`snews`: Get headlines sentiment using VADER model over time. [Source: Finnhub]\n
`snews_chart`: Display stock price and headlines sentiment using VADER model over time. [Source: Finnhub]\n
`stalker`: Gets messages from given user [Source: stocktwits].\n
diff --git a/openbb_terminal/core/sdk/models/keys_sdk_model.py b/openbb_terminal/core/sdk/models/keys_sdk_model.py
index 0572e6d3ded9..0ccee257bd40 100644
--- a/openbb_terminal/core/sdk/models/keys_sdk_model.py
+++ b/openbb_terminal/core/sdk/models/keys_sdk_model.py
@@ -42,7 +42,6 @@ class KeysRoot(Category):
`stocksera`: Set Stocksera key.\n
`tokenterminal`: Set Token Terminal key.\n
`tradier`: Set Tradier key\n
- `twitter`: Set Twitter key\n
`ultima`: Set Ultima Insights key\n
`walert`: Set Walert key\n
"""
@@ -84,6 +83,5 @@ def __init__(self):
self.stocksera = lib.keys_model.set_stocksera_key
self.tokenterminal = lib.keys_model.set_tokenterminal_key
self.tradier = lib.keys_model.set_tradier_key
- self.twitter = lib.keys_model.set_twitter_key
self.ultima = lib.keys_model.set_ultima_key
self.walert = lib.keys_model.set_walert_key
diff --git a/openbb_terminal/core/sdk/models/stocks_sdk_model.py b/openbb_terminal/core/sdk/models/stocks_sdk_model.py
index f0e4c74c4aca..193caf164e96 100644
--- a/openbb_terminal/core/sdk/models/stocks_sdk_model.py
+++ b/openbb_terminal/core/sdk/models/stocks_sdk_model.py
@@ -42,8 +42,6 @@ class StocksBehavioralAnalysis(Category):
`getdd`: Get due diligence posts from list of subreddits [Source: reddit].\n
`headlines`: Gets Sentiment analysis provided by FinBrain's API [Source: finbrain].\n
`headlines_chart`: Plots Sentiment analysis from FinBrain. Prints table if raw is True. [Source: FinBrain]\n
- `infer`: Load tweets from twitter API and analyzes using VADER.\n
- `infer_chart`: Prints Inference sentiment from past n tweets.\n
`mentions`: Get interest over time from google api [Source: google].\n
`mentions_chart`: Plots weekly bars of stock's interest over time. other users watchlist. [Source: Google].\n
`messages`: Get last messages for a given ticker [Source: stocktwits].\n
@@ -55,8 +53,6 @@ class StocksBehavioralAnalysis(Category):
`regions`: Get interest by region from google api [Source: google].\n
`regions_chart`: Plots bars of regions based on stock's interest. [Source: Google].\n
`rise`: Get top rising related queries with this stock's query [Source: google].\n
- `sentiment`: Get sentiments from symbol.\n
- `sentiment_chart`: Plots sentiments from symbol\n
`snews`: Get headlines sentiment using VADER model over time. [Source: Finnhub]\n
`snews_chart`: Display stock price and headlines sentiment using VADER model over time. [Source: Finnhub]\n
`stalker`: Gets messages from given user [Source: stocktwits].\n
@@ -74,8 +70,6 @@ def __init__(self):
self.getdd = lib.stocks_ba_reddit_model.get_due_dilligence
self.headlines = lib.stocks_ba_finbrain_model.get_sentiment
self.headlines_chart = lib.stocks_ba_finbrain_view.display_sentiment_analysis
- self.infer = lib.stocks_ba_twitter_model.load_analyze_tweets
- self.infer_chart = lib.stocks_ba_twitter_view.display_inference
self.mentions = lib.stocks_ba_google_model.get_mentions
self.mentions_chart = lib.stocks_ba_google_view.display_mentions
self.messages = lib.stocks_ba_stocktwits_model.get_messages
@@ -87,8 +81,6 @@ def __init__(self):
self.regions = lib.stocks_ba_google_model.get_regions
self.regions_chart = lib.stocks_ba_google_view.display_regions
self.rise = lib.stocks_ba_google_model.get_rise
- self.sentiment = lib.stocks_ba_twitter_model.get_sentiment
- self.sentiment_chart = lib.stocks_ba_twitter_view.display_sentiment
self.snews = lib.stocks_ba_finnhub_model.get_headlines_sentiment
self.snews_chart = (
lib.stocks_ba_finnhub_view.display_stock_price_headlines_sentiment
diff --git a/openbb_terminal/core/sdk/sdk_init.py b/openbb_terminal/core/sdk/sdk_init.py
index 0ec4b436d87c..660500334239 100644
--- a/openbb_terminal/core/sdk/sdk_init.py
+++ b/openbb_terminal/core/sdk/sdk_init.py
@@ -121,8 +121,6 @@
reddit_view as stocks_ba_reddit_view,
stocktwits_model as stocks_ba_stocktwits_model,
stocktwits_view as stocks_ba_stocktwits_view,
- twitter_model as stocks_ba_twitter_model,
- twitter_view as stocks_ba_twitter_view,
)
diff --git a/openbb_terminal/core/sdk/trail_map.csv b/openbb_terminal/core/sdk/trail_map.csv
index 33ff8830bfff..1f62a953e00e 100644
--- a/openbb_terminal/core/sdk/trail_map.csv
+++ b/openbb_terminal/core/sdk/trail_map.csv
@@ -312,7 +312,6 @@ keys.smartstake,keys_model.set_smartstake_key,
keys.stocksera,keys_model.set_stocksera_key,
keys.tokenterminal,keys_model.set_tokenterminal_key,
keys.tradier,keys_model.set_tradier_key,
-keys.twitter,keys_model.set_twitter_key,
keys.ultima,keys_model.set_ultima_key,
keys.walert,keys_model.set_walert_key,
login,sdk_session.login,
@@ -384,7 +383,6 @@ stocks.ba.bullbear,stocks_ba_stocktwits_model.get_bullbear,
stocks.ba.cnews,stocks_ba_finnhub_model.get_company_news,
stocks.ba.getdd,stocks_ba_reddit_model.get_due_dilligence,
stocks.ba.headlines,stocks_ba_finbrain_model.get_sentiment,stocks_ba_finbrain_view.display_sentiment_analysis
-stocks.ba.infer,stocks_ba_twitter_model.load_analyze_tweets,stocks_ba_twitter_view.display_inference
stocks.ba.mentions,stocks_ba_google_model.get_mentions,stocks_ba_google_view.display_mentions
stocks.ba.messages,stocks_ba_stocktwits_model.get_messages,
stocks.ba.ns,stocks_ba_news_sentiment_model.get_data,stocks_ba_news_sentiment_view.display_articles_data
@@ -393,7 +391,6 @@ stocks.ba.queries,stocks_ba_google_model.get_queries,
stocks.ba.redditsent,stocks_ba_reddit_model.get_posts_about,
stocks.ba.regions,stocks_ba_google_model.get_regions,stocks_ba_google_view.display_regions
stocks.ba.rise,stocks_ba_google_model.get_rise,
-stocks.ba.sentiment,stocks_ba_twitter_model.get_sentiment,stocks_ba_twitter_view.display_sentiment
stocks.ba.snews,stocks_ba_finnhub_model.get_headlines_sentiment,stocks_ba_finnhub_view.display_stock_price_headlines_sentiment
stocks.ba.stalker,stocks_ba_stocktwits_model.get_stalker,
stocks.ba.text_sent,stocks_ba_reddit_model.get_sentiment,
diff --git a/openbb_terminal/economy/datasets/cpi.csv b/openbb_terminal/economy/datasets/cpi.csv
index a209ce5869ef..7671215ca5a0 100644
--- a/openbb_terminal/economy/datasets/cpi.csv
+++ b/openbb_terminal/economy/datasets/cpi.csv
@@ -1,256 +1,256 @@
-title,country,frequency,units,series_id
-Consumer Price Index for Australia,australia,annual,growth_previous,CPALTT01AUA657N
-Consumer Price Index for Australia,australia,annual,growth_same,CPALTT01AUA659N
-Consumer Price Index for Australia,australia,quarterly,growth_previous,CPALTT01AUQ657N
-Consumer Price Index for Australia,australia,quarterly,growth_same,CPALTT01AUQ659N
-Consumer Price Index for Austria,austria,annual,growth_previous,CPALTT01ATA657N
-Consumer Price Index for Austria,austria,annual,growth_same,CPALTT01ATA659N
-Consumer Price Index for Austria,austria,monthly,growth_previous,CPALTT01ATM657N
-Consumer Price Index for Austria,austria,monthly,growth_same,CPALTT01ATM659N
-Consumer Price Index for Austria,austria,quarterly,growth_previous,CPALTT01ATQ657N
-Consumer Price Index for Austria,austria,quarterly,growth_same,CPALTT01ATQ659N
-Consumer Price Index for Belgium,belgium,annual,growth_previous,CPALTT01BEA657N
-Consumer Price Index for Belgium,belgium,annual,growth_same,CPALTT01BEA659N
-Consumer Price Index for Belgium,belgium,monthly,growth_previous,CPALTT01BEM657N
-Consumer Price Index for Belgium,belgium,monthly,growth_same,CPALTT01BEM659N
-Consumer Price Index for Belgium,belgium,quarterly,growth_previous,CPALTT01BEQ657N
-Consumer Price Index for Belgium,belgium,quarterly,growth_same,CPALTT01BEQ659N
-Consumer Price Index for Brazil,brazil,annual,growth_previous,CPALTT01BRA657N
-Consumer Price Index for Brazil,brazil,annual,growth_same,CPALTT01BRA659N
-Consumer Price Index for Brazil,brazil,monthly,growth_previous,CPALTT01BRM657N
-Consumer Price Index for Brazil,brazil,monthly,growth_same,CPALTT01BRM659N
-Consumer Price Index for Brazil,brazil,quarterly,growth_previous,CPALTT01BRQ657N
-Consumer Price Index for Brazil,brazil,quarterly,growth_same,CPALTT01BRQ659N
-Consumer Price Index for Canada,canada,annual,index_2015,CPALCY01CAA661N
-Consumer Price Index for Canada,canada,monthly,index_2015,CPALCY01CAM661N
-Consumer Price Index for Canada,canada,quarterly,index_2015,CPALCY01CAQ661N
-Consumer Price Index for Canada,canada,annual,growth_previous,CPALTT01CAA657N
-Consumer Price Index for Canada,canada,annual,growth_same,CPALTT01CAA659N
-Consumer Price Index for Canada,canada,monthly,growth_previous,CPALTT01CAM657N
-Consumer Price Index for Canada,canada,monthly,growth_same,CPALTT01CAM659N
-Consumer Price Index for Canada,canada,quarterly,growth_previous,CPALTT01CAQ657N
-Consumer Price Index for Canada,canada,quarterly,growth_same,CPALTT01CAQ659N
-Consumer Price Index for Chile,chile,annual,growth_previous,CPALTT01CLA657N
-Consumer Price Index for Chile,chile,annual,growth_same,CPALTT01CLA659N
-Consumer Price Index for Chile,chile,monthly,growth_previous,CPALTT01CLM657N
-Consumer Price Index for Chile,chile,monthly,growth_same,CPALTT01CLM659N
-Consumer Price Index for Chile,chile,quarterly,growth_previous,CPALTT01CLQ657N
-Consumer Price Index for Chile,chile,quarterly,growth_same,CPALTT01CLQ659N
-Consumer Price Index for China,china,annual,growth_previous,CPALTT01CNA657N
-Consumer Price Index for China,china,monthly,growth_same,CPALTT01CNM657N
-Consumer Price Index for China,china,monthly,growth_previous,CPALTT01CNM659N
-Consumer Price Index for China,china,quarterly,growth_same,CPALTT01CNQ657N
-Consumer Price Index for China,china,quarterly,growth_previous,CPALTT01CNQ659N
-Consumer Price Index for Denmark,denmark,annual,growth_same,CPALTT01DKA657N
-Consumer Price Index for Denmark,denmark,annual,growth_previous,CPALTT01DKA659N
-Consumer Price Index for Denmark,denmark,monthly,growth_same,CPALTT01DKM657N
-Consumer Price Index for Denmark,denmark,monthly,growth_previous,CPALTT01DKM659N
-Consumer Price Index for Denmark,denmark,quarterly,growth_same,CPALTT01DKQ657N
-Consumer Price Index for Denmark,denmark,quarterly,growth_previous,CPALTT01DKQ659N
-Consumer Price Index for Estonia,estonia,annual,growth_same,CPALTT01EEA657N
-Consumer Price Index for Estonia,estonia,annual,growth_previous,CPALTT01EEA659N
-Consumer Price Index for Estonia,estonia,annual,index_2015,CPALTT01EEA661N
-Consumer Price Index for Estonia,estonia,monthly,growth_previous,CPALTT01EEM657N
-Consumer Price Index for Estonia,estonia,monthly,growth_same,CPALTT01EEM659N
-Consumer Price Index for Estonia,estonia,monthly,index_2015,CPALTT01EEM661N
-Consumer Price Index for Estonia,estonia,quarterly,growth_same,CPALTT01EEQ657N
-Consumer Price Index for Estonia,estonia,quarterly,growth_previous,CPALTT01EEQ659N
-Consumer Price Index for Estonia,estonia,quarterly,index_2015,CPALTT01EEQ661N
-Consumer Price Index for Finland,finland,annual,growth_previous,CPALTT01FIA657N
-Consumer Price Index for Finland,finland,annual,growth_same,CPALTT01FIA659N
-Consumer Price Index for Finland,finland,monthly,growth_previous,CPALTT01FIM657N
-Consumer Price Index for Finland,finland,monthly,growth_same,CPALTT01FIM659N
-Consumer Price Index for Finland,finland,quarterly,growth_previous,CPALTT01FIQ657N
-Consumer Price Index for Finland,finland,quarterly,growth_same,CPALTT01FIQ659N
-Consumer Price Index for France,france,annual,growth_previous,CPALTT01FRA657N
-Consumer Price Index for France,france,annual,growth_same,CPALTT01FRA659N
-Consumer Price Index for France,france,monthly,growth_previous,CPALTT01FRM657N
-Consumer Price Index for France,france,monthly,growth_same,CPALTT01FRM659N
-Consumer Price Index for France,france,quarterly,growth_previous,CPALTT01FRQ657N
-Consumer Price Index for France,france,quarterly,growth_same,CPALTT01FRQ659N
-Consumer Price Index for Germany,germany,annual,growth_same,CPALTT01DEA657N
-Consumer Price Index for Germany,germany,annual,growth_previous,CPALTT01DEA659N
-Consumer Price Index for Germany,germany,monthly,growth_same,CPALTT01DEM657N
-Consumer Price Index for Germany,germany,monthly,growth_previous,CPALTT01DEM659N
-Consumer Price Index for Germany,germany,quarterly,growth_same,CPALTT01DEQ657N
-Consumer Price Index for Germany,germany,quarterly,growth_previous,CPALTT01DEQ659N
-Consumer Price Index for Greece,greece,annual,growth_previous,CPALTT01GRA657N
-Consumer Price Index for Greece,greece,annual,growth_same,CPALTT01GRA659N
-Consumer Price Index for Greece,greece,monthly,growth_previous,CPALTT01GRM657N
-Consumer Price Index for Greece,greece,monthly,growth_same,CPALTT01GRM659N
-Consumer Price Index for Greece,greece,quarterly,growth_previous,CPALTT01GRQ657N
-Consumer Price Index for Greece,greece,quarterly,growth_same,CPALTT01GRQ659N
-Consumer Price Index for Hungary,hungary,annual,growth_previous,CPALTT01HUA657N
-Consumer Price Index for Hungary,hungary,annual,growth_same,CPALTT01HUA659N
-Consumer Price Index for Hungary,hungary,monthly,growth_previous,CPALTT01HUM657N
-Consumer Price Index for Hungary,hungary,monthly,growth_same,CPALTT01HUM659N
-Consumer Price Index for Hungary,hungary,quarterly,growth_previous,CPALTT01HUQ657N
-Consumer Price Index for Hungary,hungary,quarterly,growth_same,CPALTT01HUQ659N
-Consumer Price Index for Iceland,iceland,annual,growth_previous,CPALTT01ISA657N
-Consumer Price Index for Iceland,iceland,annual,growth_same,CPALTT01ISA659N
-Consumer Price Index for Iceland,iceland,monthly,growth_previous,CPALTT01ISM657N
-Consumer Price Index for Iceland,iceland,monthly,growth_same,CPALTT01ISM659N
-Consumer Price Index for Iceland,iceland,quarterly,growth_previous,CPALTT01ISQ657N
-Consumer Price Index for Iceland,iceland,quarterly,growth_same,CPALTT01ISQ659N
-Consumer Price Index for India,india,annual,growth_previous,CPALTT01INA657N
-Consumer Price Index for India,india,annual,growth_same,CPALTT01INA659N
-Consumer Price Index for India,india,monthly,growth_previous,CPALTT01INM657N
-Consumer Price Index for India,india,monthly,growth_same,CPALTT01INM659N
-Consumer Price Index for India,india,quarterly,growth_previous,CPALTT01INQ657N
-Consumer Price Index for India,india,quarterly,growth_same,CPALTT01INQ659N
-Consumer Price Index for Indonesia,indonesia,annual,growth_previous,CPALTT01IDA657N
-Consumer Price Index for Indonesia,indonesia,annual,growth_same,CPALTT01IDA659N
-Consumer Price Index for Indonesia,indonesia,monthly,growth_previous,CPALTT01IDM657N
-Consumer Price Index for Indonesia,indonesia,monthly,growth_same,CPALTT01IDM659N
-Consumer Price Index for Indonesia,indonesia,quarterly,growth_previous,CPALTT01IDQ657N
-Consumer Price Index for Indonesia,indonesia,quarterly,growth_same,CPALTT01IDQ659N
-Consumer Price Index for Ireland,ireland,annual,growth_previous,CPALTT01IEA657N
-Consumer Price Index for Ireland,ireland,annual,growth_same,CPALTT01IEA659N
-Consumer Price Index for Ireland,ireland,monthly,growth_previous,CPALTT01IEM657N
-Consumer Price Index for Ireland,ireland,monthly,growth_same,CPALTT01IEM659N
-Consumer Price Index for Ireland,ireland,quarterly,growth_previous,CPALTT01IEQ657N
-Consumer Price Index for Ireland,ireland,quarterly,growth_same,CPALTT01IEQ659N
-Consumer Price Index for Israel,israel,annual,growth_previous,CPALTT01ILA657N
-Consumer Price Index for Israel,israel,annual,growth_same,CPALTT01ILA659N
-Consumer Price Index for Israel,israel,monthly,growth_previous,CPALTT01ILM657N
-Consumer Price Index for Israel,israel,monthly,growth_same,CPALTT01ILM659N
-Consumer Price Index for Israel,israel,quarterly,growth_previous,CPALTT01ILQ657N
-Consumer Price Index for Israel,israel,quarterly,growth_same,CPALTT01ILQ659N
-Consumer Price Index for Italy,italy,annual,growth_previous,CPALTT01ITA657N
-Consumer Price Index for Italy,italy,annual,growth_same,CPALTT01ITA659N
-Consumer Price Index for Italy,italy,monthly,growth_previous,CPALTT01ITM657N
-Consumer Price Index for Italy,italy,monthly,growth_same,CPALTT01ITM659N
-Consumer Price Index for Italy,italy,quarterly,growth_previous,CPALTT01ITQ657N
-Consumer Price Index for Italy,italy,quarterly,growth_same,CPALTT01ITQ659N
-Consumer Price Index for Japan,japan,monthly,index_2015,CPALCY01JPM661N
-Consumer Price Index for Japan,japan,quarterly,index_2015,CPALCY01JPQ661N
-Consumer Price Index for Japan,japan,monthly,growth_previous,CPALTT01JPM657N
-Consumer Price Index for Japan,japan,monthly,growth_same,CPALTT01JPM659N
-Consumer Price Index for Japan,japan,monthly,index_2015,CPALTT01JPM661S
-Consumer Price Index for Japan,japan,quarterly,growth_same,CPALTT01JPQ657N
-Consumer Price Index for Japan,japan,quarterly,growth_previous,CPALTT01JPQ659N
-Consumer Price Index for Japan,japan,quarterly,index_2015,CPALTT01JPQ661S
-Consumer Price Index for Luxembourg,luxembourg,annual,growth_previous,CPALTT01LUA657N
-Consumer Price Index for Luxembourg,luxembourg,annual,growth_same,CPALTT01LUA659N
-Consumer Price Index for Luxembourg,luxembourg,monthly,growth_previous,CPALTT01LUM657N
-Consumer Price Index for Luxembourg,luxembourg,monthly,growth_same,CPALTT01LUM659N
-Consumer Price Index for Luxembourg,luxembourg,quarterly,growth_previous,CPALTT01LUQ657N
-Consumer Price Index for Luxembourg,luxembourg,quarterly,growth_same,CPALTT01LUQ659N
-Consumer Price Index for Mexico,mexico,annual,index_2015,CPALCY01MXA661N
-Consumer Price Index for Mexico,mexico,monthly,index_2015,CPALCY01MXM661N
-Consumer Price Index for Mexico,mexico,quarterly,index_2015,CPALCY01MXQ661N
-Consumer Price Index for Mexico,mexico,annual,growth_previous,CPALTT01MXA657N
-Consumer Price Index for Mexico,mexico,annual,growth_same,CPALTT01MXA659N
-Consumer Price Index for Mexico,mexico,monthly,growth_previous,CPALTT01MXM657N
-Consumer Price Index for Mexico,mexico,monthly,growth_same,CPALTT01MXM659N
-Consumer Price Index for Mexico,mexico,quarterly,growth_previous,CPALTT01MXQ657N
-Consumer Price Index for Mexico,mexico,quarterly,growth_same,CPALTT01MXQ659N
-Consumer Price Index for New Zealand,new_zealand,annual,index_2015,CPALCY01NZA661N
-Consumer Price Index for New Zealand,new_zealand,quarterly,index_2015,CPALCY01NZQ661N
-Consumer Price Index for New Zealand,new_zealand,annual,growth_previous,CPALTT01NZA657N
-Consumer Price Index for New Zealand,new_zealand,annual,growth_same,CPALTT01NZA659N
-Consumer Price Index for New Zealand,new_zealand,quarterly,growth_previous,CPALTT01NZQ657N
-Consumer Price Index for New Zealand,new_zealand,quarterly,growth_same,CPALTT01NZQ659N
-Consumer Price Index for Norway,norway,annual,growth_previous,CPALTT01NOA657N
-Consumer Price Index for Norway,norway,annual,growth_same,CPALTT01NOA659N
-Consumer Price Index for Norway,norway,monthly,growth_previous,CPALTT01NOM657N
-Consumer Price Index for Norway,norway,monthly,growth_same,CPALTT01NOM659N
-Consumer Price Index for Norway,norway,quarterly,growth_previous,CPALTT01NOQ657N
-Consumer Price Index for Norway,norway,quarterly,growth_same,CPALTT01NOQ659N
-Consumer Price Index for Poland,poland,annual,growth_previous,CPALTT01PLA657N
-Consumer Price Index for Poland,poland,annual,growth_same,CPALTT01PLA659N
-Consumer Price Index for Poland,poland,monthly,growth_previous,CPALTT01PLM657N
-Consumer Price Index for Poland,poland,monthly,growth_same,CPALTT01PLM659N
-Consumer Price Index for Poland,poland,quarterly,growth_previous,CPALTT01PLQ657N
-Consumer Price Index for Poland,poland,quarterly,growth_same,CPALTT01PLQ659N
-Consumer Price Index for Portugal,portugal,annual,growth_previous,CPALTT01PTA657N
-Consumer Price Index for Portugal,portugal,annual,growth_same,CPALTT01PTA659N
-Consumer Price Index for Portugal,portugal,monthly,growth_previous,CPALTT01PTM657N
-Consumer Price Index for Portugal,portugal,monthly,growth_same,CPALTT01PTM659N
-Consumer Price Index for Portugal,portugal,quarterly,growth_previous,CPALTT01PTQ657N
-Consumer Price Index for Portugal,portugal,quarterly,growth_same,CPALTT01PTQ659N
-Consumer Price Index for Slovenia,slovenia,annual,growth_previous,CPALTT01SIA657N
-Consumer Price Index for Slovenia,slovenia,annual,growth_same,CPALTT01SIA659N
-Consumer Price Index for Slovenia,slovenia,monthly,growth_previous,CPALTT01SIM657N
-Consumer Price Index for Slovenia,slovenia,monthly,growth_same,CPALTT01SIM659N
-Consumer Price Index for Slovenia,slovenia,quarterly,growth_previous,CPALTT01SIQ657N
-Consumer Price Index for Slovenia,slovenia,quarterly,growth_same,CPALTT01SIQ659N
-Consumer Price Index for South Africa,south_africa,annual,growth_previous,CPALTT01ZAA657N
-Consumer Price Index for South Africa,south_africa,annual,growth_same,CPALTT01ZAA659N
-Consumer Price Index for South Africa,south_africa,monthly,growth_previous,CPALTT01ZAM657N
-Consumer Price Index for South Africa,south_africa,monthly,growth_same,CPALTT01ZAM659N
-Consumer Price Index for South Africa,south_africa,quarterly,growth_previous,CPALTT01ZAQ657N
-Consumer Price Index for South Africa,south_africa,quarterly,growth_same,CPALTT01ZAQ659N
-Consumer Price Index for Spain,spain,annual,growth_previous,CPALTT01ESA657N
-Consumer Price Index for Spain,spain,annual,growth_same,CPALTT01ESA659N
-Consumer Price Index for Spain,spain,monthly,growth_previous,CPALTT01ESM657N
-Consumer Price Index for Spain,spain,monthly,growth_same,CPALTT01ESM659N
-Consumer Price Index for Spain,spain,quarterly,growth_previous,CPALTT01ESQ657N
-Consumer Price Index for Spain,spain,quarterly,growth_same,CPALTT01ESQ659N
-Consumer Price Index for Sweden,sweden,annual,growth_previous,CPALTT01SEA657N
-Consumer Price Index for Sweden,sweden,annual,growth_same,CPALTT01SEA659N
-Consumer Price Index for Sweden,sweden,monthly,growth_previous,CPALTT01SEM657N
-Consumer Price Index for Sweden,sweden,monthly,growth_same,CPALTT01SEM659N
-Consumer Price Index for Sweden,sweden,quarterly,growth_previous,CPALTT01SEQ657N
-Consumer Price Index for Sweden,sweden,quarterly,growth_same,CPALTT01SEQ659N
-Consumer Price Index for Switzerland,switzerland,annual,growth_previous,CPALTT01CHA657N
-Consumer Price Index for Switzerland,switzerland,annual,growth_same,CPALTT01CHA659N
-Consumer Price Index for Switzerland,switzerland,monthly,growth_previous,CPALTT01CHM657N
-Consumer Price Index for Switzerland,switzerland,monthly,growth_same,CPALTT01CHM659N
-Consumer Price Index for Switzerland,switzerland,quarterly,growth_previous,CPALTT01CHQ657N
-Consumer Price Index for Switzerland,switzerland,quarterly,growth_same,CPALTT01CHQ659N
-Consumer Price Index for the Czech Republic,czech_republic,annual,growth_same,CPALTT01CZA657N
-Consumer Price Index for the Czech Republic,czech_republic,annual,growth_previous,CPALTT01CZA659N
-Consumer Price Index for the Czech Republic,czech_republic,monthly,growth_same,CPALTT01CZM657N
-Consumer Price Index for the Czech Republic,czech_republic,monthly,growth_previous,CPALTT01CZM659N
-Consumer Price Index for the Czech Republic,czech_republic,quarterly,growth_same,CPALTT01CZQ657N
-Consumer Price Index for the Czech Republic,czech_republic,quarterly,growth_previous,CPALTT01CZQ659N
-Consumer Price Index for the Netherlands,netherlands,annual,growth_previous,CPALTT01NLA657N
-Consumer Price Index for the Netherlands,netherlands,annual,growth_same,CPALTT01NLA659N
-Consumer Price Index for the Netherlands,netherlands,monthly,growth_previous,CPALTT01NLM657N
-Consumer Price Index for the Netherlands,netherlands,monthly,growth_same,CPALTT01NLM659N
-Consumer Price Index for the Netherlands,netherlands,quarterly,growth_previous,CPALTT01NLQ657N
-Consumer Price Index for the Netherlands,netherlands,quarterly,growth_same,CPALTT01NLQ659N
-Consumer Price Index for the Republic of Korea,korea,annual,growth_previous,CPALTT01KRA657N
-Consumer Price Index for the Republic of Korea,korea,annual,growth_same,CPALTT01KRA659N
-Consumer Price Index for the Republic of Korea,korea,monthly,growth_previous,CPALTT01KRM657N
-Consumer Price Index for the Republic of Korea,korea,monthly,growth_same,CPALTT01KRM659N
-Consumer Price Index for the Republic of Korea,korea,quarterly,growth_previous,CPALTT01KRQ657N
-Consumer Price Index for the Republic of Korea,korea,quarterly,growth_same,CPALTT01KRQ659N
-Consumer Price Index for the Russian Federation,russian_federation,monthly,growth_previous,CPALTT01RUM657N
-Consumer Price Index for the Russian Federation,russian_federation,monthly,growth_same,CPALTT01RUM659N
-Consumer Price Index for the Russian Federation,russian_federation,quarterly,growth_previous,CPALTT01RUQ657N
-Consumer Price Index for the Russian Federation,russian_federation,quarterly,growth_same,CPALTT01RUQ659N
-Consumer Price Index for the Slovak Republic,slovak_republic,annual,growth_previous,CPALTT01SKA657N
-Consumer Price Index for the Slovak Republic,slovak_republic,annual,growth_same,CPALTT01SKA659N
-Consumer Price Index for the Slovak Republic,slovak_republic,monthly,growth_previous,CPALTT01SKM657N
-Consumer Price Index for the Slovak Republic,slovak_republic,monthly,growth_same,CPALTT01SKM659N
-Consumer Price Index for the Slovak Republic,slovak_republic,quarterly,growth_previous,CPALTT01SKQ657N
-Consumer Price Index for the Slovak Republic,slovak_republic,quarterly,growth_same,CPALTT01SKQ659N
-Consumer Price Index for the United Kingdom,the united kingdom,annual,growth_previous,CPALTT01GBA657N
-Consumer Price Index for the United Kingdom,united_kingdom,annual,growth_same,CPALTT01GBA659N
-Consumer Price Index for the United Kingdom,united_kingdom,monthly,growth_previous,CPALTT01GBM657N
-Consumer Price Index for the United Kingdom,united_kingdom,monthly,growth_same,CPALTT01GBM659N
-Consumer Price Index for the United Kingdom,united_kingdom,quarterly,growth_previous,CPALTT01GBQ657N
-Consumer Price Index for the United Kingdom,united_kingdom,quarterly,growth_same,CPALTT01GBQ659N
-Consumer Price Index for the United States,united_states,annual,index_2015,CPALCY01USA661N
-Consumer Price Index for the United States,united_states,monthly,index_2015,CPALCY01USM661N
-Consumer Price Index for the United States,united_states,quarterly,index_2015,CPALCY01USQ661N
-Consumer Price Index for the United States,united_states,annual,growth_previous,CPALTT01USA657N
-Consumer Price Index for the United States,united_states,annual,growth_same,CPALTT01USA659N
-Consumer Price Index for the United States,united_states,annual,index_2015,CPALTT01USA661S
-Consumer Price Index for the United States,united_states,monthly,growth_previous,CPALTT01USM657N
-Consumer Price Index for the United States,united_states,monthly,growth_same,CPALTT01USM659N
-Consumer Price Index for the United States,united_states,monthly,index_2015,CPALTT01USM661S
-Consumer Price Index for the United States,united_states,quarterly,growth_previous,CPALTT01USQ657N
-Consumer Price Index for the United States,united_states,quarterly,growth_same,CPALTT01USQ659N
-Consumer Price Index for the United States,united_states,quarterly,index_2015,CPALTT01USQ661S
-Consumer Price Index for Turkey,turkey,annual,index_2015,CPALCY01TRA661N
-Consumer Price Index for Turkey,turkey,monthly,index_2015,CPALCY01TRM661N
-Consumer Price Index for Turkey,turkey,quarterly,index_2015,CPALCY01TRQ661N
-Consumer Price Index for Turkey,turkey,annual,growth_previous,CPALTT01TRA657N
-Consumer Price Index for Turkey,turkey,annual,growth_same,CPALTT01TRA659N
-Consumer Price Index for Turkey,turkey,monthly,growth_previous,CPALTT01TRM657N
-Consumer Price Index for Turkey,turkey,monthly,growth_same,CPALTT01TRM659N
-Consumer Price Index for Turkey,turkey,quarterly,growth_previous,CPALTT01TRQ657N
+title,country,frequency,units,series_id
+Consumer Price Index for Australia,australia,annual,growth_previous,CPALTT01AUA657N
+Consumer Price Index for Australia,australia,annual,growth_same,CPALTT01AUA659N
+Consumer Price Index for Australia,australia,quarterly,growth_previous,CPALTT01AUQ657N
+Consumer Price Index for Australia,australia,quarterly,growth_same,CPALTT01AUQ659N
+Consumer Price Index for Austria,austria,annual,growth_previous,CPALTT01ATA657N
+Consumer Price Index for Austria,austria,annual,growth_same,CPALTT01ATA659N
+Consumer Price Index for Austria,austria,monthly,growth_previous,CPALTT01ATM657N
+Consumer Price Index for Austria,austria,monthly,growth_same,CPALTT01ATM659N
+Consumer Price Index for Austria,austria,quarterly,growth_previous,CPALTT01ATQ657N
+Consumer Price Index for Austria,austria,quarterly,growth_same,CPALTT01ATQ659N
+Consumer Price Index for Belgium,belgium,annual,growth_previous,CPALTT01BEA657N
+Consumer Price Index for Belgium,belgium,annual,growth_same,CPALTT01BEA659N
+Consumer Price Index for Belgium,belgium,monthly,growth_previous,CPALTT01BEM657N
+Consumer Price Index for Belgium,belgium,monthly,growth_same,CPALTT01BEM659N
+Consumer Price Index for Belgium,belgium,quarterly,growth_previous,CPALTT01BEQ657N
+Consumer Price Index for Belgium,belgium,quarterly,growth_same,CPALTT01BEQ659N
+Consumer Price Index for Brazil,brazil,annual,growth_previous,CPALTT01BRA657N
+Consumer Price Index for Brazil,brazil,annual,growth_same,CPALTT01BRA659N
+Consumer Price Index for Brazil,brazil,monthly,growth_previous,CPALTT01BRM657N
+Consumer Price Index for Brazil,brazil,monthly,growth_same,CPALTT01BRM659N
+Consumer Price Index for Brazil,brazil,quarterly,growth_previous,CPALTT01BRQ657N
+Consumer Price Index for Brazil,brazil,quarterly,growth_same,CPALTT01BRQ659N
+Consumer Price Index for Canada,canada,annual,index_2015,CPALCY01CAA661N
+Consumer Price Index for Canada,canada,monthly,index_2015,CPALCY01CAM661N
+Consumer Price Index for Canada,canada,quarterly,index_2015,CPALCY01CAQ661N
+Consumer Price Index for Canada,canada,annual,growth_previous,CPALTT01CAA657N
+Consumer Price Index for Canada,canada,annual,growth_same,CPALTT01CAA659N
+Consumer Price Index for Canada,canada,monthly,growth_previous,CPALTT01CAM657N
+Consumer Price Index for Canada,canada,monthly,growth_same,CPALTT01CAM659N
+Consumer Price Index for Canada,canada,quarterly,growth_previous,CPALTT01CAQ657N
+Consumer Price Index for Canada,canada,quarterly,growth_same,CPALTT01CAQ659N
+Consumer Price Index for Chile,chile,annual,growth_previous,CPALTT01CLA657N
+Consumer Price Index for Chile,chile,annual,growth_same,CPALTT01CLA659N
+Consumer Price Index for Chile,chile,monthly,growth_previous,CPALTT01CLM657N
+Consumer Price Index for Chile,chile,monthly,growth_same,CPALTT01CLM659N
+Consumer Price Index for Chile,chile,quarterly,growth_previous,CPALTT01CLQ657N
+Consumer Price Index for Chile,chile,quarterly,growth_same,CPALTT01CLQ659N
+Consumer Price Index for China,china,annual,growth_previous,CPALTT01CNA657N
+Consumer Price Index for China,china,monthly,growth_previous,CPALTT01CNM657N
+Consumer Price Index for China,china,monthly,growth_same,CPALTT01CNM659N
+Consumer Price Index for China,china,quarterly,growth_previous,CPALTT01CNQ657N
+Consumer Price Index for China,china,quarterly,growth_same,CPALTT01CNQ659N
+Consumer Price Index for Denmark,denmark,annual,growth_previous,CPALTT01DKA657N
+Consumer Price Index for Denmark,denmark,annual,growth_same,CPALTT01DKA659N
+Consumer Price Index for Denmark,denmark,monthly,growth_previous,CPALTT01DKM657N
+Consumer Price Index for Denmark,denmark,monthly,growth_same,CPALTT01DKM659N
+Consumer Price Index for Denmark,denmark,quarterly,growth_previous,CPALTT01DKQ657N
+Consumer Price Index for Denmark,denmark,quarterly,growth_same,CPALTT01DKQ659N
+Consumer Price Index for Estonia,estonia,annual,growth_previous,CPALTT01EEA657N
+Consumer Price Index for Estonia,estonia,annual,growth_same,CPALTT01EEA659N
+Consumer Price Index for Estonia,estonia,annual,index_2015,CPALTT01EEA661N
+Consumer Price Index for Estonia,estonia,monthly,growth_previous,CPALTT01EEM657N
+Consumer Price Index for Estonia,estonia,monthly,growth_same,CPALTT01EEM659N
+Consumer Price Index for Estonia,estonia,monthly,index_2015,CPALTT01EEM661N
+Consumer Price Index for Estonia,estonia,quarterly,growth_previous,CPALTT01EEQ657N
+Consumer Price Index for Estonia,estonia,quarterly,growth_same,CPALTT01EEQ659N
+Consumer Price Index for Estonia,estonia,quarterly,index_2015,CPALTT01EEQ661N
+Consumer Price Index for Finland,finland,annual,growth_previous,CPALTT01FIA657N
+Consumer Price Index for Finland,finland,annual,growth_same,CPALTT01FIA659N
+Consumer Price Index for Finland,finland,monthly,growth_previous,CPALTT01FIM657N
+Consumer Price Index for Finland,finland,monthly,growth_same,CPALTT01FIM659N
+Consumer Price Index for Finland,finland,quarterly,growth_previous,CPALTT01FIQ657N
+Consumer Price Index for Finland,finland,quarterly,growth_same,CPALTT01FIQ659N
+Consumer Price Index for France,france,annual,growth_previous,CPALTT01FRA657N
+Consumer Price Index for France,france,annual,growth_same,CPALTT01FRA659N
+Consumer Price Index for France,france,monthly,growth_previous,CPALTT01FRM657N
+Consumer Price Index for France,france,monthly,growth_same,CPALTT01FRM659N
+Consumer Price Index for France,france,quarterly,growth_previous,CPALTT01FRQ657N
+Consumer Price Index for France,france,quarterly,growth_same,CPALTT01FRQ659N
+Consumer Price Index for Germany,germany,annual,growth_previous,CPALTT01DEA657N
+Consumer Price Index for Germany,germany,annual,growth_same,CPALTT01DEA659N
+Consumer Price Index for Germany,germany,monthly,growth_previous,CPALTT01DEM657N
+Consumer Price Index for Germany,germany,monthly,growth_same,CPALTT01DEM659N
+Consumer Price Index for Germany,germany,quarterly,growth_previous,CPALTT01DEQ657N
+Consumer Price Index for Germany,germany,quarterly,growth_same,CPALTT01DEQ659N
+Consumer Price Index for Greece,greece,annual,growth_previous,CPALTT01GRA657N
+Consumer Price Index for Greece,greece,annual,growth_same,CPALTT01GRA659N
+Consumer Price Index for Greece,greece,monthly,growth_previous,CPALTT01GRM657N
+Consumer Price Index for Greece,greece,monthly,growth_same,CPALTT01GRM659N
+Consumer Price Index for Greece,greece,quarterly,growth_previous,CPALTT01GRQ657N
+Consumer Price Index for Greece,greece,quarterly,growth_same,CPALTT01GRQ659N
+Consumer Price Index for Hungary,hungary,annual,growth_previous,CPALTT01HUA657N
+Consumer Price Index for Hungary,hungary,annual,growth_same,CPALTT01HUA659N
+Consumer Price Index for Hungary,hungary,monthly,growth_previous,CPALTT01HUM657N
+Consumer Price Index for Hungary,hungary,monthly,growth_same,CPALTT01HUM659N
+Consumer Price Index for Hungary,hungary,quarterly,growth_previous,CPALTT01HUQ657N
+Consumer Price Index for Hungary,hungary,quarterly,growth_same,CPALTT01HUQ659N
+Consumer Price Index for Iceland,iceland,annual,growth_previous,CPALTT01ISA657N
+Consumer Price Index for Iceland,iceland,annual,growth_same,CPALTT01ISA659N
+Consumer Price Index for Iceland,iceland,monthly,growth_previous,CPALTT01ISM657N
+Consumer Price Index for Iceland,iceland,monthly,growth_same,CPALTT01ISM659N
+Consumer Price Index for Iceland,iceland,quarterly,growth_previous,CPALTT01ISQ657N
+Consumer Price Index for Iceland,iceland,quarterly,growth_same,CPALTT01ISQ659N
+Consumer Price Index for India,india,annual,growth_previous,CPALTT01INA657N
+Consumer Price Index for India,india,annual,growth_same,CPALTT01INA659N
+Consumer Price Index for India,india,monthly,growth_previous,CPALTT01INM657N
+Consumer Price Index for India,india,monthly,growth_same,CPALTT01INM659N
+Consumer Price Index for India,india,quarterly,growth_previous,CPALTT01INQ657N
+Consumer Price Index for India,india,quarterly,growth_same,CPALTT01INQ659N
+Consumer Price Index for Indonesia,indonesia,annual,growth_previous,CPALTT01IDA657N
+Consumer Price Index for Indonesia,indonesia,annual,growth_same,CPALTT01IDA659N
+Consumer Price Index for Indonesia,indonesia,monthly,growth_previous,CPALTT01IDM657N
+Consumer Price Index for Indonesia,indonesia,monthly,growth_same,CPALTT01IDM659N
+Consumer Price Index for Indonesia,indonesia,quarterly,growth_previous,CPALTT01IDQ657N
+Consumer Price Index for Indonesia,indonesia,quarterly,growth_same,CPALTT01IDQ659N
+Consumer Price Index for Ireland,ireland,annual,growth_previous,CPALTT01IEA657N
+Consumer Price Index for Ireland,ireland,annual,growth_same,CPALTT01IEA659N
+Consumer Price Index for Ireland,ireland,monthly,growth_previous,CPALTT01IEM657N
+Consumer Price Index for Ireland,ireland,monthly,growth_same,CPALTT01IEM659N
+Consumer Price Index for Ireland,ireland,quarterly,growth_previous,CPALTT01IEQ657N
+Consumer Price Index for Ireland,ireland,quarterly,growth_same,CPALTT01IEQ659N
+Consumer Price Index for Israel,israel,annual,growth_previous,CPALTT01ILA657N
+Consumer Price Index for Israel,israel,annual,growth_same,CPALTT01ILA659N
+Consumer Price Index for Israel,israel,monthly,growth_previous,CPALTT01ILM657N
+Consumer Price Index for Israel,israel,monthly,growth_same,CPALTT01ILM659N
+Consumer Price Index for Israel,israel,quarterly,growth_previous,CPALTT01ILQ657N
+Consumer Price Index for Israel,israel,quarterly,growth_same,CPALTT01ILQ659N
+Consumer Price Index for Italy,italy,annual,growth_previous,CPALTT01ITA657N
+Consumer Price Index for Italy,italy,annual,growth_same,CPALTT01ITA659N
+Consumer Price Index for Italy,italy,monthly,growth_previous,CPALTT01ITM657N
+Consumer Price Index for Italy,italy,monthly,growth_same,CPALTT01ITM659N
+Consumer Price Index for Italy,italy,quarterly,growth_previous,CPALTT01ITQ657N
+Consumer Price Index for Italy,italy,quarterly,growth_same,CPALTT01ITQ659N
+Consumer Price Index for Japan,japan,monthly,index_2015,CPALCY01JPM661N
+Consumer Price Index for Japan,japan,quarterly,index_2015,CPALCY01JPQ661N
+Consumer Price Index for Japan,japan,monthly,growth_previous,CPALTT01JPM657N
+Consumer Price Index for Japan,japan,monthly,growth_same,CPALTT01JPM659N
+Consumer Price Index for Japan,japan,monthly,index_2015,CPALTT01JPM661S
+Consumer Price Index for Japan,japan,quarterly,growth_previous,CPALTT01JPQ657N
+Consumer Price Index for Japan,japan,quarterly,growth_same,CPALTT01JPQ659N
+Consumer Price Index for Japan,japan,quarterly,index_2015,CPALTT01JPQ661S
+Consumer Price Index for Luxembourg,luxembourg,annual,growth_previous,CPALTT01LUA657N
+Consumer Price Index for Luxembourg,luxembourg,annual,growth_same,CPALTT01LUA659N
+Consumer Price Index for Luxembourg,luxembourg,monthly,growth_previous,CPALTT01LUM657N
+Consumer Price Index for Luxembourg,luxembourg,monthly,growth_same,CPALTT01LUM659N
+Consumer Price Index for Luxembourg,luxembourg,quarterly,growth_previous,CPALTT01LUQ657N
+Consumer Price Index for Luxembourg,luxembourg,quarterly,growth_same,CPALTT01LUQ659N
+Consumer Price Index for Mexico,mexico,annual,index_2015,CPALCY01MXA661N
+Consumer Price Index for Mexico,mexico,monthly,index_2015,CPALCY01MXM661N
+Consumer Price Index for Mexico,mexico,quarterly,index_2015,CPALCY01MXQ661N
+Consumer Price Index for Mexico,mexico,annual,growth_previous,CPALTT01MXA657N
+Consumer Price Index for Mexico,mexico,annual,growth_same,CPALTT01MXA659N
+Consumer Price Index for Mexico,mexico,monthly,growth_previous,CPALTT01MXM657N
+Consumer Price Index for Mexico,mexico,monthly,growth_same,CPALTT01MXM659N
+Consumer Price Index for Mexico,mexico,quarterly,growth_previous,CPALTT01MXQ657N
+Consumer Price Index for Mexico,mexico,quarterly,growth_same,CPALTT01MXQ659N
+Consumer Price Index for New Zealand,new_zealand,annual,index_2015,CPALCY01NZA661N
+Consumer Price Index for New Zealand,new_zealand,quarterly,index_2015,CPALCY01NZQ661N
+Consumer Price Index for New Zealand,new_zealand,annual,growth_previous,CPALTT01NZA657N
+Consumer Price Index for New Zealand,new_zealand,annual,growth_same,CPALTT01NZA659N
+Consumer Price Index for New Zealand,new_zealand,quarterly,growth_previous,CPALTT01NZQ657N
+Consumer Price Index for New Zealand,new_zealand,quarterly,growth_same,CPALTT01NZQ659N
+Consumer Price Index for Norway,norway,annual,growth_previous,CPALTT01NOA657N
+Consumer Price Index for Norway,norway,annual,growth_same,CPALTT01NOA659N
+Consumer Price Index for Norway,norway,monthly,growth_previous,CPALTT01NOM657N
+Consumer Price Index for Norway,norway,monthly,growth_same,CPALTT01NOM659N
+Consumer Price Index for Norway,norway,quarterly,growth_previous,CPALTT01NOQ657N
+Consumer Price Index for Norway,norway,quarterly,growth_same,CPALTT01NOQ659N
+Consumer Price Index for Poland,poland,annual,growth_previous,CPALTT01PLA657N
+Consumer Price Index for Poland,poland,annual,growth_same,CPALTT01PLA659N
+Consumer Price Index for Poland,poland,monthly,growth_previous,CPALTT01PLM657N
+Consumer Price Index for Poland,poland,monthly,growth_same,CPALTT01PLM659N
+Consumer Price Index for Poland,poland,quarterly,growth_previous,CPALTT01PLQ657N
+Consumer Price Index for Poland,poland,quarterly,growth_same,CPALTT01PLQ659N
+Consumer Price Index for Portugal,portugal,annual,growth_previous,CPALTT01PTA657N
+Consumer Price Index for Portugal,portugal,annual,growth_same,CPALTT01PTA659N
+Consumer Price Index for Portugal,portugal,monthly,growth_previous,CPALTT01PTM657N
+Consumer Price Index for Portugal,portugal,monthly,growth_same,CPALTT01PTM659N
+Consumer Price Index for Portugal,portugal,quarterly,growth_previous,CPALTT01PTQ657N
+Consumer Price Index for Portugal,portugal,quarterly,growth_same,CPALTT01PTQ659N
+Consumer Price Index for Slovenia,slovenia,annual,growth_previous,CPALTT01SIA657N
+Consumer Price Index for Slovenia,slovenia,annual,growth_same,CPALTT01SIA659N
+Consumer Price Index for Slovenia,slovenia,monthly,growth_previous,CPALTT01SIM657N
+Consumer Price Index for Slovenia,slovenia,monthly,growth_same,CPALTT01SIM659N
+Consumer Price Index for Slovenia,slovenia,quarterly,growth_previous,CPALTT01SIQ657N
+Consumer Price Index for Slovenia,slovenia,quarterly,growth_same,CPALTT01SIQ659N
+Consumer Price Index for South Africa,south_africa,annual,growth_previous,CPALTT01ZAA657N
+Consumer Price Index for South Africa,south_africa,annual,growth_same,CPALTT01ZAA659N
+Consumer Price Index for South Africa,south_africa,monthly,growth_previous,CPALTT01ZAM657N
+Consumer Price Index for South Africa,south_africa,monthly,growth_same,CPALTT01ZAM659N
+Consumer Price Index for South Africa,south_africa,quarterly,growth_previous,CPALTT01ZAQ657N
+Consumer Price Index for South Africa,south_africa,quarterly,growth_same,CPALTT01ZAQ659N
+Consumer Price Index for Spain,spain,annual,growth_previous,CPALTT01ESA657N
+Consumer Price Index for Spain,spain,annual,growth_same,CPALTT01ESA659N
+Consumer Price Index for Spain,spain,monthly,growth_previous,CPALTT01ESM657N
+Consumer Price Index for Spain,spain,monthly,growth_same,CPALTT01ESM659N
+Consumer Price Index for Spain,spain,quarterly,growth_previous,CPALTT01ESQ657N
+Consumer Price Index for Spain,spain,quarterly,growth_same,CPALTT01ESQ659N
+Consumer Price Index for Sweden,sweden,annual,growth_previous,CPALTT01SEA657N
+Consumer Price Index for Sweden,sweden,annual,growth_same,CPALTT01SEA659N
+Consumer Price Index for Sweden,sweden,monthly,growth_previous,CPALTT01SEM657N
+Consumer Price Index for Sweden,sweden,monthly,growth_same,CPALTT01SEM659N
+Consumer Price Index for Sweden,sweden,quarterly,growth_previous,CPALTT01SEQ657N
+Consumer Price Index for Sweden,sweden,quarterly,growth_same,CPALTT01SEQ659N
+Consumer Price Index for Switzerland,switzerland,annual,growth_previous,CPALTT01CHA657N
+Consumer Price Index for Switzerland,switzerland,annual,growth_same,CPALTT01CHA659N
+Consumer Price Index for Switzerland,switzerland,monthly,growth_previous,CPALTT01CHM657N
+Consumer Price Index for Switzerland,switzerland,monthly,growth_same,CPALTT01CHM659N
+Consumer Price Index for Switzerland,switzerland,quarterly,growth_previous,CPALTT01CHQ657N
+Consumer Price Index for Switzerland,switzerland,quarterly,growth_same,CPALTT01CHQ659N
+Consumer Price Index for the Czech Republic,czech_republic,annual,growth_previous,CPALTT01CZA657N
+Consumer Price Index for the Czech Republic,czech_republic,annual,growth_same,CPALTT01CZA659N
+Consumer Price Index for the Czech Republic,czech_republic,monthly,growth_previous,CPALTT01CZM657N
+Consumer Price Index for the Czech Republic,czech_republic,monthly,growth_same,CPALTT01CZM659N
+Consumer Price Index for the Czech Republic,czech_republic,quarterly,growth_previous,CPALTT01CZQ657N
+Consumer Price Index for the Czech Republic,czech_republic,quarterly,growth_same,CPALTT01CZQ659N
+Consumer Price Index for the Netherlands,netherlands,annual,growth_previous,CPALTT01NLA657N
+Consumer Price Index for the Netherlands,netherlands,annual,growth_same,CPALTT01NLA659N
+Consumer Price Index for the Netherlands,netherlands,monthly,growth_previous,CPALTT01NLM657N
+Consumer Price Index for the Netherlands,netherlands,monthly,growth_same,CPALTT01NLM659N
+Consumer Price Index for the Netherlands,netherlands,quarterly,growth_previous,CPALTT01NLQ657N
+Consumer Price Index for the Netherlands,netherlands,quarterly,growth_same,CPALTT01NLQ659N
+Consumer Price Index for the Republic of Korea,korea,annual,growth_previous,CPALTT01KRA657N
+Consumer Price Index for the Republic of Korea,korea,annual,growth_same,CPALTT01KRA659N
+Consumer Price Index for the Republic of Korea,korea,monthly,growth_previous,CPALTT01KRM657N
+Consumer Price Index for the Republic of Korea,korea,monthly,growth_same,CPALTT01KRM659N
+Consumer Price Index for the Republic of Korea,korea,quarterly,growth_previous,CPALTT01KRQ657N
+Consumer Price Index for the Republic of Korea,korea,quarterly,growth_same,CPALTT01KRQ659N
+Consumer Price Index for the Russian Federation,russian_federation,monthly,growth_previous,CPALTT01RUM657N
+Consumer Price Index for the Russian Federation,russian_federation,monthly,growth_same,CPALTT01RUM659N
+Consumer Price Index for the Russian Federation,russian_federation,quarterly,growth_previous,CPALTT01RUQ657N
+Consumer Price Index for the Russian Federation,russian_federation,quarterly,growth_same,CPALTT01RUQ659N
+Consumer Price Index for the Slovak Republic,slovak_republic,annual,growth_previous,CPALTT01SKA657N
+Consumer Price Index for the Slovak Republic,slovak_republic,annual,growth_same,CPALTT01SKA659N
+Consumer Price Index for the Slovak Republic,slovak_republic,monthly,growth_previous,CPALTT01SKM657N
+Consumer Price Index for the Slovak Republic,slovak_republic,monthly,growth_same,CPALTT01SKM659N
+Consumer Price Index for the Slovak Republic,slovak_republic,quarterly,growth_previous,CPALTT01SKQ657N
+Consumer Price Index for the Slovak Republic,slovak_republic,quarterly,growth_same,CPALTT01SKQ659N
+Consumer Price Index for the United Kingdom,the united kingdom,annual,growth_previous,CPALTT01GBA657N
+Consumer Price Index for the United Kingdom,united_kingdom,annual,growth_same,CPALTT01GBA659N
+Consumer Price Index for the United Kingdom,united_kingdom,monthly,growth_previous,CPALTT01GBM657N
+Consumer Price Index for the United Kingdom,united_kingdom,monthly,growth_same,CPALTT01GBM659N
+Consumer Price Index for the United Kingdom,united_kingdom,quarterly,growth_previous,CPALTT01GBQ657N
+Consumer Price Index for the United Kingdom,united_kingdom,quarterly,growth_same,CPALTT01GBQ659N
+Consumer Price Index for the United States,united_states,annual,index_2015,CPALCY01USA661N
+Consumer Price Index for the United States,united_states,monthly,index_2015,CPALCY01USM661N
+Consumer Price Index for the United States,united_states,quarterly,index_2015,CPALCY01USQ661N
+Consumer Price Index for the United States,united_states,annual,growth_previous,CPALTT01USA657N
+Consumer Price Index for the United States,united_states,annual,growth_same,CPALTT01USA659N
+Consumer Price Index for the United States,united_states,annual,index_2015,CPALTT01USA661S
+Consumer Price Index for the United States,united_states,monthly,growth_previous,CPALTT01USM657N
+Consumer Price Index for the United States,united_states,monthly,growth_same,CPALTT01USM659N
+Consumer Price Index for the United States,united_states,monthly,index_2015,CPALTT01USM661S
+Consumer Price Index for the United States,united_states,quarterly,growth_previous,CPALTT01USQ657N
+Consumer Price Index for the United States,united_states,quarterly,growth_same,CPALTT01USQ659N
+Consumer Price Index for the United States,united_states,quarterly,index_2015,CPALTT01USQ661S
+Consumer Price Index for Turkey,turkey,annual,index_2015,CPALCY01TRA661N
+Consumer Price Index for Turkey,turkey,monthly,index_2015,CPALCY01TRM661N
+Consumer Price Index for Turkey,turkey,quarterly,index_2015,CPALCY01TRQ661N
+Consumer Price Index for Turkey,turkey,annual,growth_previous,CPALTT01TRA657N
+Consumer Price Index for Turkey,turkey,annual,growth_same,CPALTT01TRA659N
+Consumer Price Index for Turkey,turkey,monthly,growth_previous,CPALTT01TRM657N
+Consumer Price Index for Turkey,turkey,monthly,growth_same,CPALTT01TRM659N
+Consumer Price Index for Turkey,turkey,quarterly,growth_previous,CPALTT01TRQ657N
Consumer Price Index for Turkey,turkey,quarterly,growth_same,CPALTT01TRQ659N
\ No newline at end of file
diff --git a/openbb_terminal/keys_controller.py b/openbb_terminal/keys_controller.py
index 69c94595a53d..976475b934a0 100644
--- a/openbb_terminal/keys_controller.py
+++ b/openbb_terminal/keys_controller.py
@@ -577,52 +577,6 @@ def call_reddit(self, other_args: List[str]):
show_output=True,
)
- @log_start_end(log=logger)
- def call_twitter(self, other_args: List[str]):
- """Process twitter command"""
- parser = argparse.ArgumentParser(
- add_help=False,
- formatter_class=argparse.ArgumentDefaultsHelpFormatter,
- prog="twitter",
- description="Set Twitter API key.",
- )
- parser.add_argument(
- "-k",
- "--key",
- type=str,
- dest="key",
- help="Key",
- required="-h" not in other_args and "--help" not in other_args,
- )
- parser.add_argument(
- "-s",
- "--secret",
- type=str,
- dest="secret",
- help="Secret key",
- required="-h" not in other_args and "--help" not in other_args,
- )
- parser.add_argument(
- "-t",
- "--token",
- type=str,
- dest="token",
- help="Bearer token",
- required="-h" not in other_args and "--help" not in other_args,
- )
- if not other_args:
- console.print("For your API Key, visit: https://developer.twitter.com")
- return
- ns_parser = self.parse_simple_args(parser, other_args)
- if ns_parser:
- self.status_dict["twitter"] = keys_model.set_twitter_key(
- key=ns_parser.key,
- secret=ns_parser.secret,
- access_token=ns_parser.token,
- persist=True,
- show_output=True,
- )
-
@log_start_end(log=logger)
def call_rh(self, other_args: List[str]):
"""Process rh command"""
diff --git a/openbb_terminal/keys_model.py b/openbb_terminal/keys_model.py
index 12d6347f6048..5582e0aea3a1 100644
--- a/openbb_terminal/keys_model.py
+++ b/openbb_terminal/keys_model.py
@@ -64,7 +64,6 @@
"cmc": "COINMARKETCAP",
"finnhub": "FINNHUB",
"reddit": "REDDIT",
- "twitter": "TWITTER",
"rh": "ROBINHOOD",
"degiro": "DEGIRO",
"oanda": "OANDA",
@@ -1160,100 +1159,6 @@ def check_bitquery_key(show_output: bool = False) -> str:
return str(status)
-def set_twitter_key(
- key: str,
- secret: str,
- access_token: str,
- persist: bool = False,
- show_output: bool = False,
-) -> str:
- """Set Twitter key
-
- Parameters
- ----------
- key: str
- API key
- secret: str
- API secret
- access_token: str
- API token
- persist: bool, optional
- If False, api key change will be contained to where it was changed. For example, a Jupyter notebook session.
- If True, api key change will be global, i.e. it will affect terminal environment variables.
- By default, False.
- show_output: bool, optional
- Display status string or not. By default, False.
-
- Returns
- -------
- str
- Status of key set
-
- Examples
- --------
- >>> from openbb_terminal.sdk import openbb
- >>> openbb.keys.twitter(
- key="example_key",
- secret="example_secret",
- access_token="example_access_token"
- )
- """
- handle_credential("API_TWITTER_KEY", key, persist)
- handle_credential("API_TWITTER_SECRET_KEY", secret, persist)
- handle_credential("API_TWITTER_BEARER_TOKEN", access_token, persist)
-
- return check_twitter_key(show_output)
-
-
-def check_twitter_key(show_output: bool = False) -> str:
- """Check Twitter key
-
- Parameters
- ----------
- show_output: bool, optional
- Display status string or not. By default, False.
-
- Returns
- -------
- str
- Status of key set
- """
-
- if show_output:
- console.print("Checking status...")
-
- current_user = get_current_user()
- if current_user.credentials.API_TWITTER_BEARER_TOKEN == "REPLACE_ME":
- status = KeyStatus.NOT_DEFINED
- else:
- params = {
- "query": "(\\$AAPL) (lang:en)",
- "max_results": "10",
- "tweet.fields": "created_at,lang",
- }
- r = request(
- "https://api.twitter.com/2/tweets/search/recent",
- params=params, # type: ignore
- headers={
- "authorization": "Bearer "
- + current_user.credentials.API_TWITTER_BEARER_TOKEN
- },
- )
- if r.status_code == 200:
- status = KeyStatus.DEFINED_TEST_PASSED
- elif r.status_code in [401, 403]:
- logger.warning("Twitter key defined, test failed")
- status = KeyStatus.DEFINED_TEST_FAILED
- else:
- logger.warning("Twitter key defined, test failed")
- status = KeyStatus.DEFINED_TEST_INCONCLUSIVE
-
- if show_output:
- console.print(status.colorize())
-
- return str(status)
-
-
def set_rh_key(
username: str,
password: str,
diff --git a/openbb_terminal/miscellaneous/integration_tests_scripts/stocks/test_ba.openbb b/openbb_terminal/miscellaneous/integration_tests_scripts/stocks/test_ba.openbb
index bdb897524169..df8fe96274ec 100644
--- a/openbb_terminal/miscellaneous/integration_tests_scripts/stocks/test_ba.openbb
+++ b/openbb_terminal/miscellaneous/integration_tests_scripts/stocks/test_ba.openbb
@@ -2,29 +2,21 @@ stocks
ba
load ${ticker=aapl}
headlines
-headlines --raw
wsb
-wsb --new
-watchlist
-popular -l 5 -n 10
-spacc
-spac
+popular
getdd
redditsent
trending
-stalker -l 3
+stalker
bullbear
messages
-infer
-sentiment -d 3 -l 2
mentions
regions
interest -w yolo
queries
rise
-jcdr
-jctr
snews
+ns
help
reset
exit
diff --git a/openbb_terminal/miscellaneous/models/all_api_keys.json b/openbb_terminal/miscellaneous/models/all_api_keys.json
index d9abe8d6dc97..cac66c9c54ea 100644
--- a/openbb_terminal/miscellaneous/models/all_api_keys.json
+++ b/openbb_terminal/miscellaneous/models/all_api_keys.json
@@ -65,24 +65,6 @@
"link": "https://polygon.io",
"markdown": "Go to: https://polygon.io\n\n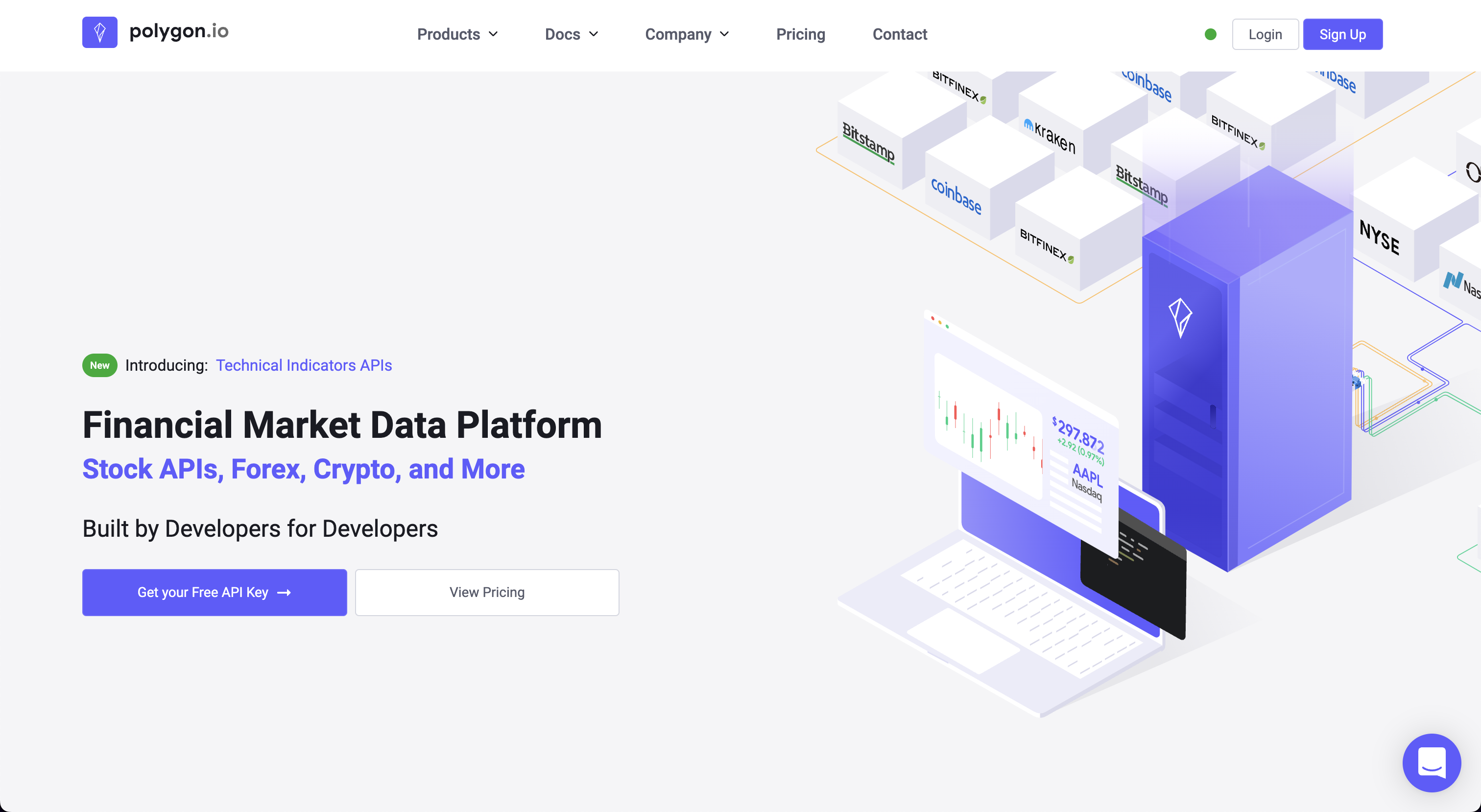\n\nClick on, \"Get your Free API Key\".\n\n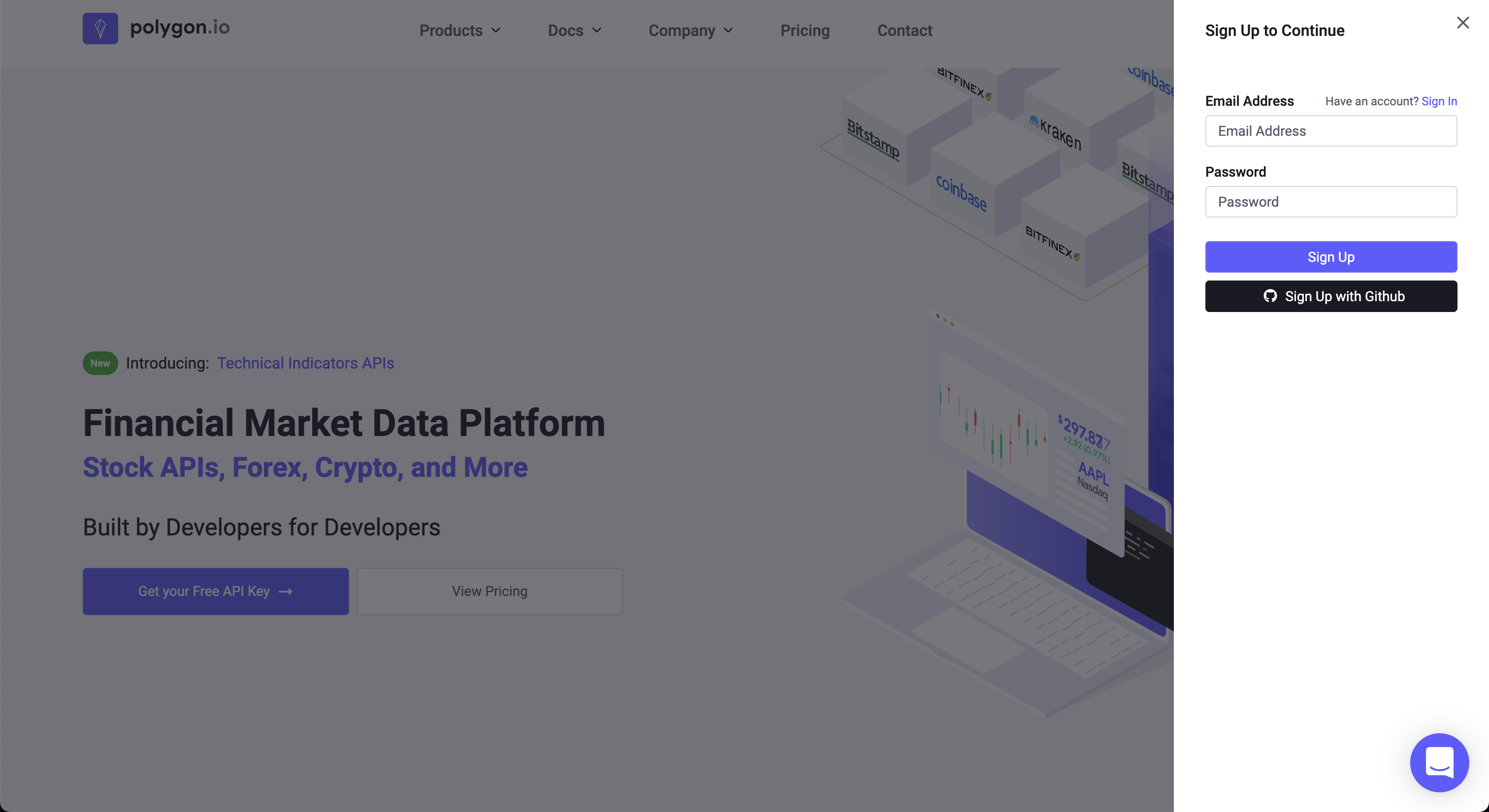\n\nAfter signing up, the API Key is found at the bottom of the account dashboard page.\n\n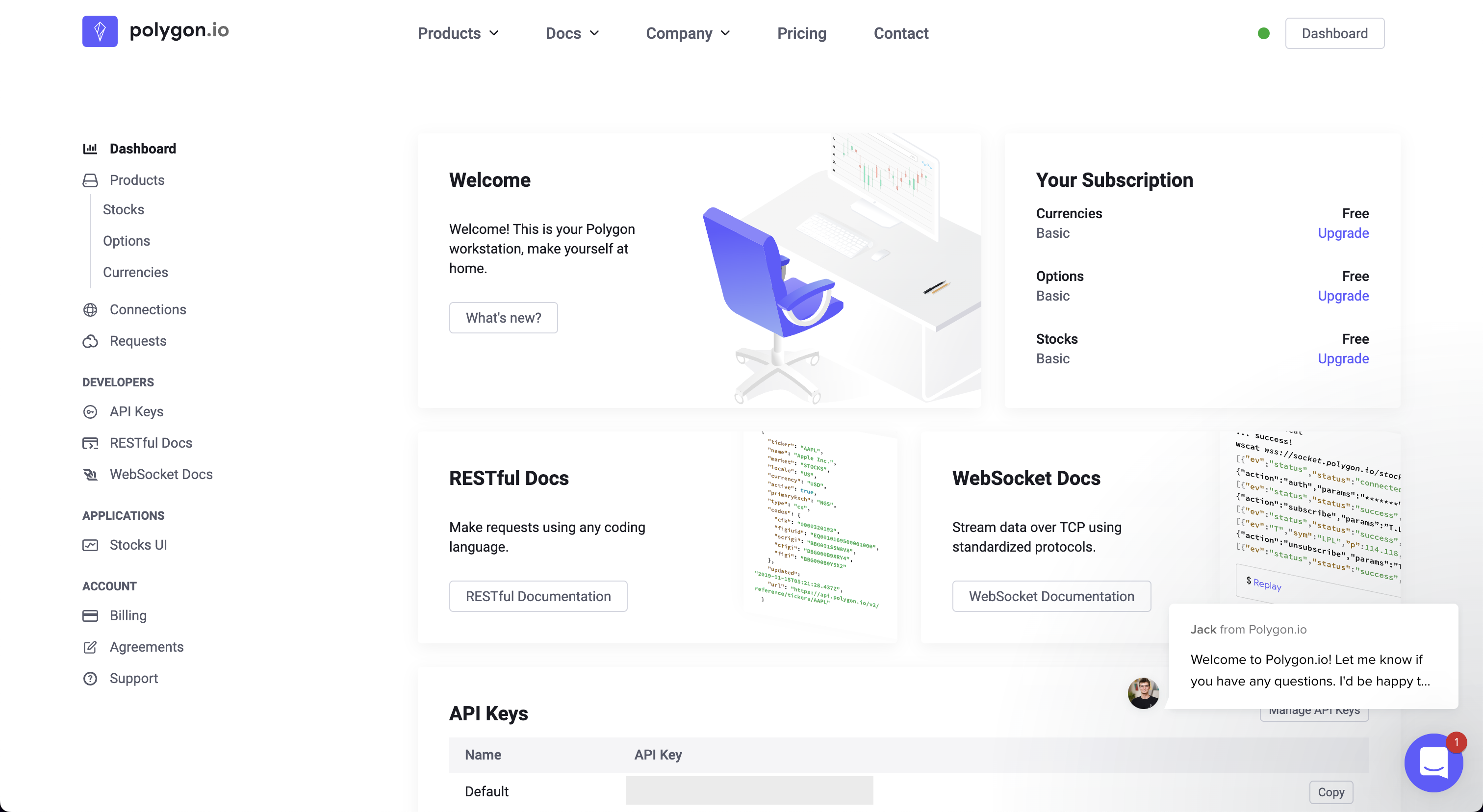"
},
- {
- "name": "API_TWITTER_KEY",
- "source": "Twitter key",
- "link": "https://developer.twitter.com",
- "markdown": "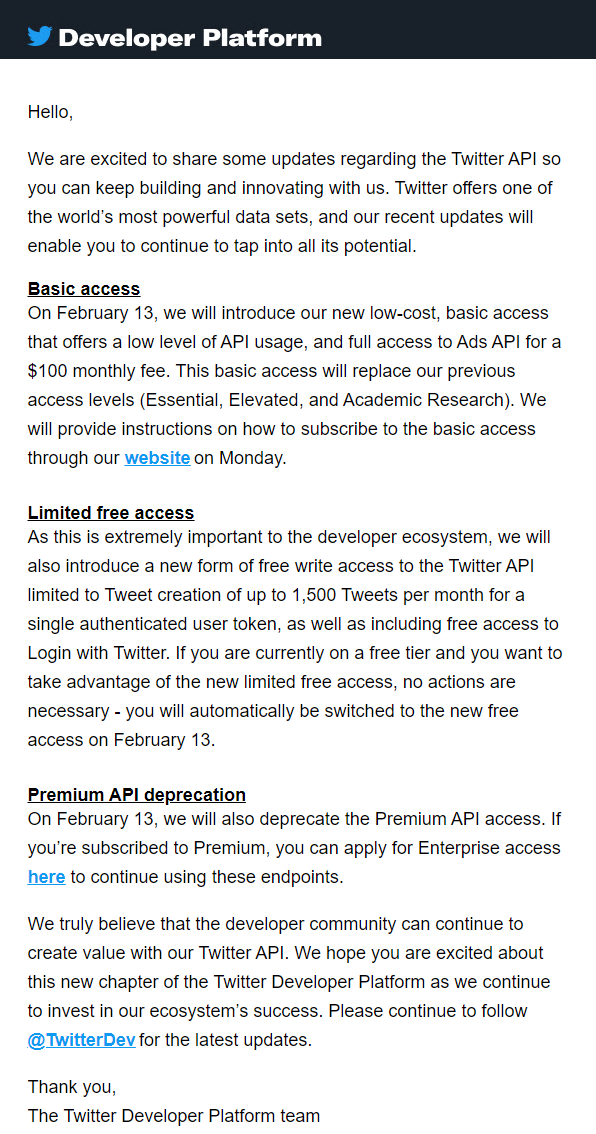"
- },
- {
- "name": "API_TWITTER_SECRET_KEY",
- "source": "Twitter secret",
- "link": "https://developer.twitter.com",
- "markdown": "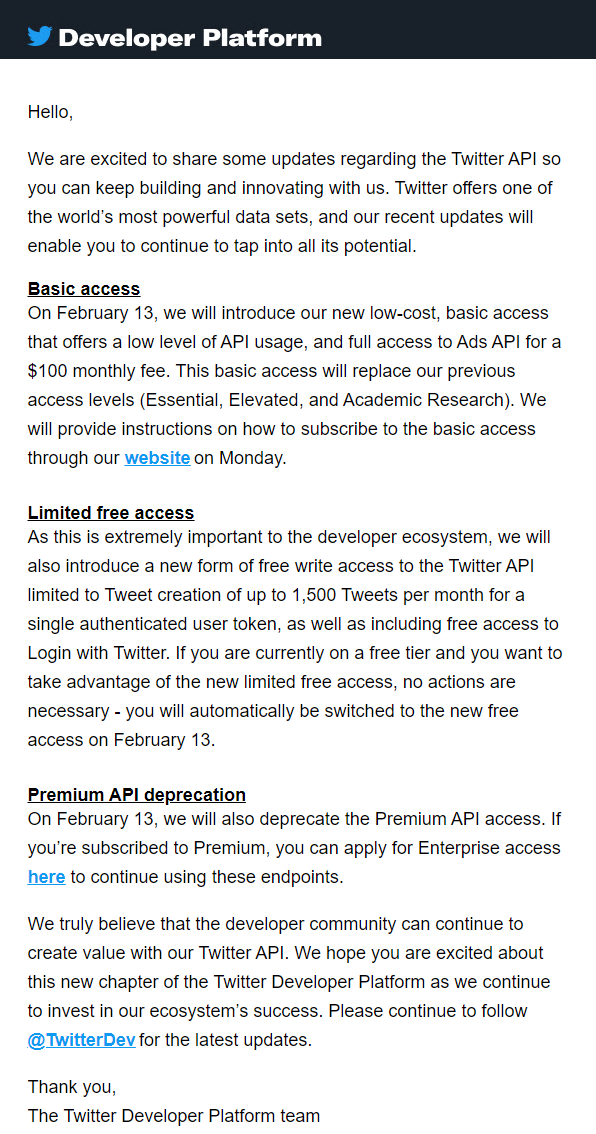"
- },
- {
- "name": "API_TWITTER_BEARER_TOKEN",
- "source": "Twitter token",
- "link": "https://developer.twitter.com",
- "markdown": "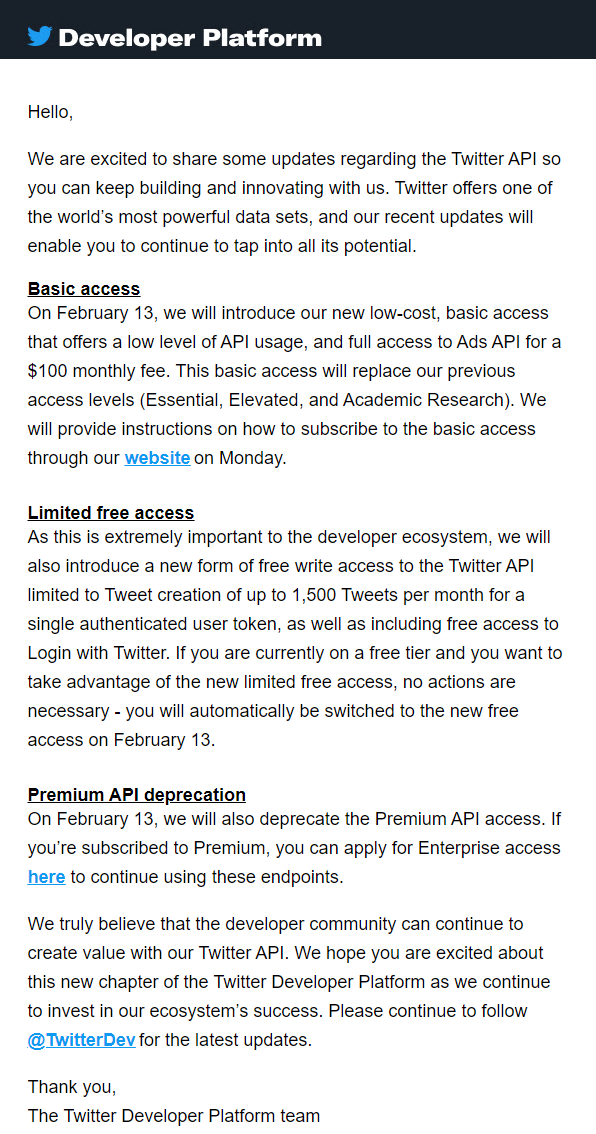"
- },
{
"name": "API_FRED_KEY",
"source": "FRED",
diff --git a/openbb_terminal/miscellaneous/models/hub_credentials.json b/openbb_terminal/miscellaneous/models/hub_credentials.json
index c0275c172bbb..2370ee7b71d3 100644
--- a/openbb_terminal/miscellaneous/models/hub_credentials.json
+++ b/openbb_terminal/miscellaneous/models/hub_credentials.json
@@ -32,8 +32,5 @@
"API_REDDIT_USERNAME": "",
"API_REDDIT_USER_AGENT": "",
"API_REDDIT_PASSWORD": "",
- "API_TWITTER_KEY": "",
- "API_TWITTER_SECRET_KEY": "",
- "API_TWITTER_BEARER_TOKEN": "",
"API_DAPPRADAR_KEY": ""
}
diff --git a/openbb_terminal/sdk.py b/openbb_terminal/sdk.py
index 73165a284649..1990c434c1ef 100644
--- a/openbb_terminal/sdk.py
+++ b/openbb_terminal/sdk.py
@@ -438,7 +438,6 @@ def keys(self):
`stocksera`: Set Stocksera key.\n
`tokenterminal`: Set Token Terminal key.\n
`tradier`: Set Tradier key\n
- `twitter`: Set Twitter key\n
`ultima`: Set Ultima Insights key\n
`walert`: Set Walert key\n
"""
diff --git a/openbb_terminal/stocks/behavioural_analysis/ba_controller.py b/openbb_terminal/stocks/behavioural_analysis/ba_controller.py
index 00953436ee6c..547c5f23897c 100644
--- a/openbb_terminal/stocks/behavioural_analysis/ba_controller.py
+++ b/openbb_terminal/stocks/behavioural_analysis/ba_controller.py
@@ -13,7 +13,6 @@
google_view,
reddit_view,
stocktwits_view,
- twitter_view,
)
from openbb_terminal.core.session.current_user import get_current_user
from openbb_terminal.custom_prompt_toolkit import NestedCompleter
@@ -21,7 +20,6 @@
from openbb_terminal.helper_funcs import (
EXPORT_BOTH_RAW_DATA_AND_FIGURES,
EXPORT_ONLY_RAW_DATA_ALLOWED,
- check_int_range,
check_non_negative,
check_positive,
valid_date,
@@ -53,8 +51,6 @@ class BehaviouralAnalysisController(StockBaseController):
"messages",
"trending",
"stalker",
- "infer",
- "sentiment",
"mentions",
"regions",
"queries",
@@ -102,8 +98,6 @@ def print_help(self):
mt.add_cmd("stalker")
mt.add_cmd("bullbear", self.ticker)
mt.add_cmd("messages", self.ticker)
- mt.add_cmd("infer", self.ticker)
- mt.add_cmd("sentiment", self.ticker)
mt.add_cmd("mentions", self.ticker)
mt.add_cmd("regions", self.ticker)
mt.add_cmd("interest", self.ticker)
@@ -673,106 +667,6 @@ def call_rise(self, other_args: List[str]):
else:
console.print("No ticker loaded. Please load using 'load '\n")
- @log_start_end(log=logger)
- def call_infer(self, other_args: List[str]):
- """Process infer command."""
- parser = argparse.ArgumentParser(
- add_help=False,
- formatter_class=argparse.ArgumentDefaultsHelpFormatter,
- prog="infer",
- description="""
- Print quick sentiment inference from last tweets that contain the ticker.
- This model splits the text into character-level tokens and uses vader sentiment analysis.
- [Source: Twitter]
- """,
- )
- parser.add_argument(
- "-l",
- "--limit",
- action="store",
- dest="limit",
- type=check_int_range(10, 100),
- default=100,
- help="limit of latest tweets to infer from.",
- )
- if other_args and "-" not in other_args[0][0]:
- other_args.insert(0, "-l")
- ns_parser = self.parse_known_args_and_warn(
- parser, other_args, EXPORT_ONLY_RAW_DATA_ALLOWED
- )
- if ns_parser:
- if self.ticker:
- twitter_view.display_inference(
- symbol=self.ticker,
- limit=ns_parser.limit,
- export=ns_parser.export,
- sheet_name=" ".join(ns_parser.sheet_name)
- if ns_parser.sheet_name
- else None,
- )
- else:
- console.print("No ticker loaded. Please load using 'load '\n")
-
- @log_start_end(log=logger)
- def call_sentiment(self, other_args: List[str]):
- """Process sentiment command."""
- parser = argparse.ArgumentParser(
- add_help=False,
- formatter_class=argparse.ArgumentDefaultsHelpFormatter,
- prog="sentiment",
- description="""
- Plot in-depth sentiment predicted from tweets from last days
- that contain pre-defined ticker. [Source: Twitter]
- """,
- )
- # in reality this argument could be 100, but after testing it takes too long
- # to compute which may not be acceptable
- # TODO: use https://github.com/twintproject/twint instead of twitter API
- parser.add_argument(
- "-l",
- "--limit",
- action="store",
- dest="limit",
- type=check_int_range(10, 62),
- default=15,
- help="limit of tweets to extract per hour.",
- )
- parser.add_argument(
- "-d",
- "--days",
- action="store",
- dest="n_days_past",
- type=check_int_range(1, 6),
- default=6,
- help="number of days in the past to extract tweets.",
- )
- parser.add_argument(
- "-c",
- "--compare",
- action="store_true",
- dest="compare",
- help="show corresponding change in stock price",
- )
- if other_args and "-" not in other_args[0][0]:
- other_args.insert(0, "-l")
- ns_parser = self.parse_known_args_and_warn(
- parser, other_args, EXPORT_BOTH_RAW_DATA_AND_FIGURES
- )
- if ns_parser:
- if self.ticker:
- twitter_view.display_sentiment(
- symbol=self.ticker,
- n_tweets=ns_parser.limit,
- n_days_past=ns_parser.n_days_past,
- compare=ns_parser.compare,
- export=ns_parser.export,
- sheet_name=" ".join(ns_parser.sheet_name)
- if ns_parser.sheet_name
- else None,
- )
- else:
- console.print("No ticker loaded. Please load using 'load '\n")
-
@log_start_end(log=logger)
def call_headlines(self, other_args: List[str]):
"""Process finbrain command."""
diff --git a/tests/openbb_terminal/common/behavioural_analysis/test_twitter_model.py b/tests/openbb_terminal/common/behavioural_analysis/test_twitter_model.py
deleted file mode 100644
index e69de29bb2d1..000000000000
diff --git a/tests/openbb_terminal/common/behavioural_analysis/test_twitter_view.py b/tests/openbb_terminal/common/behavioural_analysis/test_twitter_view.py
deleted file mode 100644
index e69de29bb2d1..000000000000
diff --git a/tests/openbb_terminal/stocks/behavioural_analysis/test_ba_controller.py b/tests/openbb_terminal/stocks/behavioural_analysis/test_ba_controller.py
index 888a0ac69367..c966d950db74 100644
--- a/tests/openbb_terminal/stocks/behavioural_analysis/test_ba_controller.py
+++ b/tests/openbb_terminal/stocks/behavioural_analysis/test_ba_controller.py
@@ -297,27 +297,6 @@ def test_call_func_expect_queue(expected_queue, queue, func):
limit=5,
),
),
- (
- "call_infer",
- ["--limit=20", "--export=csv"],
- "twitter_view.display_inference",
- [],
- dict(symbol="MOCK_TICKER", limit=20, export="csv", sheet_name=None),
- ),
- (
- "call_sentiment",
- ["--limit=20", "--days=2", "--compare", "--export=csv"],
- "twitter_view.display_sentiment",
- [],
- dict(
- symbol="MOCK_TICKER",
- n_tweets=20,
- n_days_past=2,
- compare=True,
- export="csv",
- sheet_name=None,
- ),
- ),
(
"call_mentions",
["--start=2020-12-01", "--export=csv"],
@@ -422,8 +401,6 @@ def test_call_func(
"call_messages",
"call_trending",
"call_stalker",
- "call_infer",
- "call_sentiment",
"call_mentions",
"call_regions",
"call_queries",
@@ -456,8 +433,6 @@ def test_call_func_no_parser(func, mocker):
"func",
[
"call_headlines",
- "call_sentiment",
- "call_infer",
"call_rise",
"call_queries",
"call_regions",
diff --git a/tests/openbb_terminal/stocks/behavioural_analysis/txt/test_ba_controller/test_print_help.txt b/tests/openbb_terminal/stocks/behavioural_analysis/txt/test_ba_controller/test_print_help.txt
index e2a9a232e0bc..e342867786e1 100644
--- a/tests/openbb_terminal/stocks/behavioural_analysis/txt/test_ba_controller/test_print_help.txt
+++ b/tests/openbb_terminal/stocks/behavioural_analysis/txt/test_ba_controller/test_print_help.txt
@@ -12,8 +12,6 @@ Ticker: TSLA
stalker stalk stocktwits user's last messages [Stocktwits]
bullbear estimate quick sentiment from last 30 messages on board [Stocktwits]
messages output up to the 30 last messages on the board [Stocktwits]
- infer infer about stock's sentiment from latest tweets [Twitter]
- sentiment in-depth sentiment prediction from tweets over time [Twitter]
mentions interest over time based on stock's mentions [Google]
regions regions that show highest interest in stock [Google]
interest interest over time of sentences versus stock price [Google]
diff --git a/tests/openbb_terminal/test_keys_controller.py b/tests/openbb_terminal/test_keys_controller.py
index 10c2de4d5cf4..8af59ae4f4dd 100644
--- a/tests/openbb_terminal/test_keys_controller.py
+++ b/tests/openbb_terminal/test_keys_controller.py
@@ -148,12 +148,6 @@ def test_call_reddit(other):
controller.call_reddit(other)
-@pytest.mark.vcr
-@pytest.mark.parametrize("other", [[], ["-k", "1234", "-s", "4567", "-t", "890"]])
-def test_call_twitter(other):
- controller.call_twitter(other)
-
-
@pytest.mark.vcr
@pytest.mark.parametrize("other", [[], ["-u", "1234", "-p", "4567"]])
def test_call_rh(other):
diff --git a/tests/openbb_terminal/test_keys_model.py b/tests/openbb_terminal/test_keys_model.py
index 7d230a13c200..f5b2883e3dd3 100644
--- a/tests/openbb_terminal/test_keys_model.py
+++ b/tests/openbb_terminal/test_keys_model.py
@@ -367,34 +367,6 @@ def test_set_bitquery_key(args: List[str], persist: bool, show_output: bool, moc
mock_check.assert_called_once_with(show_output)
-@pytest.mark.vcr
-@pytest.mark.parametrize(
- "args, persist, show_output",
- [
- (
- ["test_key", "test_secret", "test_access_token"],
- False,
- True,
- ),
- (
- ["test_key", "test_secret", "test_access_token"],
- False,
- False,
- ),
- ],
-)
-def test_set_twitter_key(args: List[str], persist: bool, show_output: bool, mocker):
- mock_check = mocker.patch("openbb_terminal.keys_model.check_twitter_key")
- keys_model.set_twitter_key(
- key=args[0],
- secret=args[1],
- access_token=args[2],
- persist=persist,
- show_output=show_output,
- )
- mock_check.assert_called_once_with(show_output)
-
-
@pytest.mark.vcr
@pytest.mark.parametrize(
"args, persist, show_output",
diff --git a/website/content/terminal/usage/guides/api-keys.md b/website/content/terminal/usage/guides/api-keys.md
index d15f8792fd51..30f712e3cb88 100644
--- a/website/content/terminal/usage/guides/api-keys.md
+++ b/website/content/terminal/usage/guides/api-keys.md
@@ -2,7 +2,7 @@
title: Setting API Keys
sidebar_position: 1
description: API (Application Programming Interface) keys are access credentials for accessing data from a particular source. Learn how to set, manage, and access data APIs for the OpenBB Terminal.
-keywords: [api, keys, api keys, openbb terminal, data provider, data, free, alpha vantage, fred, iex, twitter, degiro, binance, coinglass, polygon, intrinio, sdk, alphavantage, bitquery, coinbase, databento, finnhub, FRED, github, glassnode, iex cloud, news API, robinhood, santiment, shroomdk, token terminal, tradier, twitter, whale alert]
+keywords: [api, keys, api keys, openbb terminal, data provider, data, free, alpha vantage, fred, iex, degiro, binance, coinglass, polygon, intrinio, sdk, alphavantage, bitquery, coinbase, databento, finnhub, FRED, github, glassnode, iex cloud, news API, robinhood, santiment, shroomdk, token terminal, tradier, whale alert]
---
import HeadTitle from '@site/src/components/General/HeadTitle.tsx';