diff --git a/packages/block-library/src/gallery/index.js b/packages/block-library/src/gallery/index.js
index 137090eaf339b..b7fe92407cbec 100644
--- a/packages/block-library/src/gallery/index.js
+++ b/packages/block-library/src/gallery/index.js
@@ -17,12 +17,12 @@ import { G, Path, SVG } from '@wordpress/components';
*/
import { default as edit, defaultColumnsNumber, pickRelevantMediaFiles } from './edit';
+// Attributes are to be saved as JSON in the block boundary for easier
+// server-side access.
const blockAttributes = {
images: {
type: 'array',
default: [],
- source: 'query',
- selector: 'ul.wp-block-gallery .blocks-gallery-item',
query: {
url: {
source: 'attribute',
@@ -64,6 +64,50 @@ const blockAttributes = {
},
};
+// Identical schema, but wherein `images` are not saved in the block boundary,
+// and instead are sourced in the block's inner HTML.
+const deprecatedBlockAttributes = {
+ ...blockAttributes,
+ images: {
+ ...blockAttributes.images,
+ source: 'query',
+ selector: 'ul.wp-block-gallery .blocks-gallery-item',
+ },
+};
+
+function save( { attributes } ) {
+ const { images, columns = defaultColumnsNumber( attributes ), imageCrop, linkTo } = attributes;
+ return (
+
+ { images.map( ( image ) => {
+ let href;
+
+ switch ( linkTo ) {
+ case 'media':
+ href = image.url;
+ break;
+ case 'attachment':
+ href = image.link;
+ break;
+ }
+
+ const img =
;
+
+ return (
+ -
+
+ { href ? { img } : img }
+ { image.caption && image.caption.length > 0 && (
+
+ ) }
+
+
+ );
+ } ) }
+
+ );
+}
+
export const name = 'core/gallery';
export const settings = {
@@ -164,42 +208,15 @@ export const settings = {
edit,
- save( { attributes } ) {
- const { images, columns = defaultColumnsNumber( attributes ), imageCrop, linkTo } = attributes;
- return (
-
- { images.map( ( image ) => {
- let href;
-
- switch ( linkTo ) {
- case 'media':
- href = image.url;
- break;
- case 'attachment':
- href = image.link;
- break;
- }
-
- const img =
;
-
- return (
- -
-
- { href ? { img } : img }
- { image.caption && image.caption.length > 0 && (
-
- ) }
-
-
- );
- } ) }
-
- );
- },
+ save,
deprecated: [
{
- attributes: blockAttributes,
+ attributes: deprecatedBlockAttributes,
+ save,
+ },
+ {
+ attributes: deprecatedBlockAttributes,
save( { attributes } ) {
const { images, columns = defaultColumnsNumber( attributes ), imageCrop, linkTo } = attributes;
return (
@@ -235,9 +252,9 @@ export const settings = {
},
{
attributes: {
- ...blockAttributes,
+ ...deprecatedBlockAttributes,
images: {
- ...blockAttributes.images,
+ ...deprecatedBlockAttributes.images,
selector: 'div.wp-block-gallery figure.blocks-gallery-image img',
},
align: {
diff --git a/packages/block-library/src/image/index.js b/packages/block-library/src/image/index.js
index f7f6e17f6b66c..7e5a9c918d198 100644
--- a/packages/block-library/src/image/index.js
+++ b/packages/block-library/src/image/index.js
@@ -31,26 +31,16 @@ export const name = 'core/image';
const blockAttributes = {
url: {
type: 'string',
- source: 'attribute',
- selector: 'img',
- attribute: 'src',
},
alt: {
type: 'string',
- source: 'attribute',
- selector: 'img',
- attribute: 'alt',
default: '',
},
caption: {
- source: 'html',
- selector: 'figcaption',
+ type: 'string',
},
href: {
type: 'string',
- source: 'attribute',
- selector: 'figure > a',
- attribute: 'href',
},
id: {
type: 'number',
@@ -70,6 +60,37 @@ const blockAttributes = {
},
linkTarget: {
type: 'string',
+ },
+};
+
+const deprecatedBlockAttributes = {
+ ...blockAttributes,
+ url: {
+ ...blockAttributes.url,
+ source: 'attribute',
+ selector: 'img',
+ attribute: 'src',
+ },
+ alt: {
+ ...blockAttributes.alt,
+ source: 'attribute',
+ selector: 'img',
+ attribute: 'alt',
+ },
+ caption: {
+ ...blockAttributes.caption,
+ type: undefined,
+ source: 'html',
+ selector: 'figcaption',
+ },
+ href: {
+ ...blockAttributes.href,
+ source: 'attribute',
+ selector: 'figure > a',
+ attribute: 'href',
+ },
+ linkTarget: {
+ ...blockAttributes.linkTarget,
source: 'attribute',
selector: 'figure > a',
attribute: 'target',
@@ -99,6 +120,48 @@ const schema = {
},
};
+function save( { attributes } ) {
+ const { url, alt, caption, align, href, width, height, id, linkTarget } = attributes;
+
+ const classes = classnames( {
+ [ `align${ align }` ]: align,
+ 'is-resized': width || height,
+ } );
+
+ const image = (
+
+ );
+
+ const figure = (
+
+ { href ? { image } : image }
+ { ! RichText.isEmpty( caption ) && }
+
+ );
+
+ if ( 'left' === align || 'right' === align || 'center' === align ) {
+ return (
+
+
+ { figure }
+
+
+ );
+ }
+
+ return (
+
+ { figure }
+
+ );
+}
+
export const settings = {
title: __( 'Image' ),
@@ -212,51 +275,15 @@ export const settings = {
edit,
- save( { attributes } ) {
- const { url, alt, caption, align, href, width, height, id, linkTarget } = attributes;
-
- const classes = classnames( {
- [ `align${ align }` ]: align,
- 'is-resized': width || height,
- } );
-
- const image = (
-
- );
-
- const figure = (
-
- { href ? { image } : image }
- { ! RichText.isEmpty( caption ) && }
-
- );
-
- if ( 'left' === align || 'right' === align || 'center' === align ) {
- return (
-
-
- { figure }
-
-
- );
- }
-
- return (
-
- { figure }
-
- );
- },
+ save,
deprecated: [
{
- attributes: blockAttributes,
+ attributes: deprecatedBlockAttributes,
+ save,
+ },
+ {
+ attributes: deprecatedBlockAttributes,
save( { attributes } ) {
const { url, alt, caption, align, href, width, height, id } = attributes;
@@ -284,7 +311,7 @@ export const settings = {
},
},
{
- attributes: blockAttributes,
+ attributes: deprecatedBlockAttributes,
save( { attributes } ) {
const { url, alt, caption, align, href, width, height, id } = attributes;
@@ -307,7 +334,7 @@ export const settings = {
},
},
{
- attributes: blockAttributes,
+ attributes: deprecatedBlockAttributes,
save( { attributes } ) {
const { url, alt, caption, align, href, width, height } = attributes;
const extraImageProps = width || height ? { width, height } : {};
diff --git a/test/integration/full-content/fixtures/core__gallery.html b/test/integration/full-content/fixtures/core__gallery.html
index 5e48c7e66351f..8670368046b38 100644
--- a/test/integration/full-content/fixtures/core__gallery.html
+++ b/test/integration/full-content/fixtures/core__gallery.html
@@ -1,4 +1,4 @@
-
+
-
diff --git a/test/integration/full-content/fixtures/core__gallery.parsed.json b/test/integration/full-content/fixtures/core__gallery.parsed.json
index 3d80657c7f204..c26086e14adbe 100644
--- a/test/integration/full-content/fixtures/core__gallery.parsed.json
+++ b/test/integration/full-content/fixtures/core__gallery.parsed.json
@@ -1,7 +1,7 @@
[
{
"blockName": "core/gallery",
- "attrs": {},
+ "attrs": {"images":[{"url":"https://cldup.com/uuUqE_dXzy.jpg","alt":"title","caption":""},{"url":"http://google.com/hi.png","alt":"title","caption":""}]},
"innerBlocks": [],
"innerHTML": "\n
\n\t- \n\t\t\n\t\t\t
\n\t\t\n\t \n\t- \n\t\t\n\t\t\t
\n\t\t\n\t \n
\n"
},
diff --git a/test/integration/full-content/fixtures/core__gallery.serialized.html b/test/integration/full-content/fixtures/core__gallery.serialized.html
index 5bf6ce819f976..6f62a010f5717 100644
--- a/test/integration/full-content/fixtures/core__gallery.serialized.html
+++ b/test/integration/full-content/fixtures/core__gallery.serialized.html
@@ -1,3 +1,3 @@
-
+
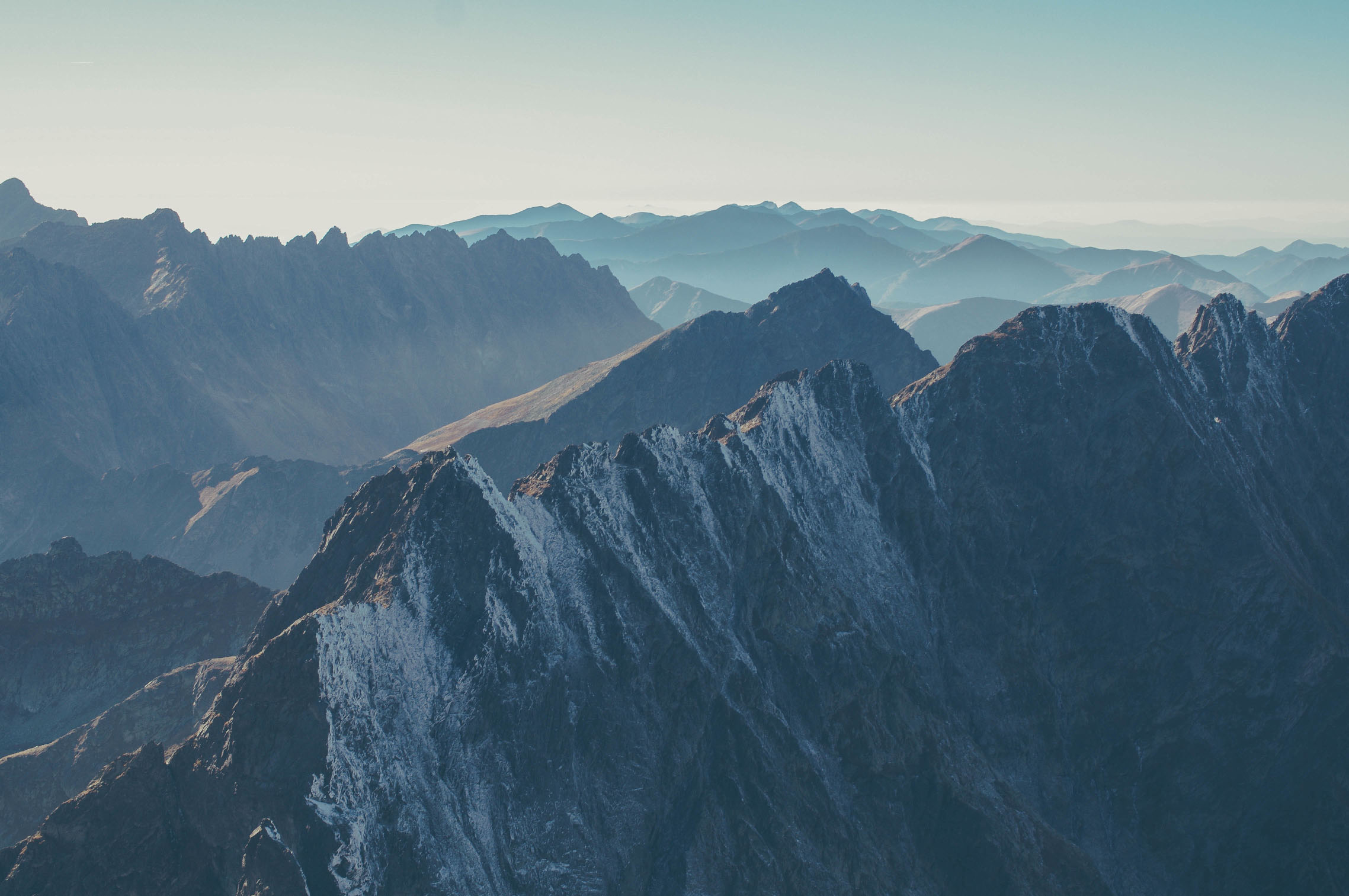

diff --git a/test/integration/full-content/fixtures/core__gallery__columns.html b/test/integration/full-content/fixtures/core__gallery__columns.html
index cf1f1bb43fa3f..822f4f068de03 100644
--- a/test/integration/full-content/fixtures/core__gallery__columns.html
+++ b/test/integration/full-content/fixtures/core__gallery__columns.html
@@ -1,4 +1,4 @@
-
+
-
diff --git a/test/integration/full-content/fixtures/core__gallery__columns.parsed.json b/test/integration/full-content/fixtures/core__gallery__columns.parsed.json
index 44faed907a8fc..1fa05927c2d47 100644
--- a/test/integration/full-content/fixtures/core__gallery__columns.parsed.json
+++ b/test/integration/full-content/fixtures/core__gallery__columns.parsed.json
@@ -2,6 +2,10 @@
{
"blockName": "core/gallery",
"attrs": {
+ "images": [
+ {"url":"https://cldup.com/uuUqE_dXzy.jpg","alt":"title","caption":""},
+ {"url":"http://google.com/hi.png","alt":"title","caption":""}
+ ],
"columns": 1
},
"innerBlocks": [],
diff --git a/test/integration/full-content/fixtures/core__gallery__columns.serialized.html b/test/integration/full-content/fixtures/core__gallery__columns.serialized.html
index 183e484ec42b6..1b3daadad3c7b 100644
--- a/test/integration/full-content/fixtures/core__gallery__columns.serialized.html
+++ b/test/integration/full-content/fixtures/core__gallery__columns.serialized.html
@@ -1,3 +1,3 @@
-
+
diff --git a/test/integration/full-content/fixtures/core__image.html b/test/integration/full-content/fixtures/core__image.html
index eda663561a38b..c07c11ebc74db 100644
--- a/test/integration/full-content/fixtures/core__image.html
+++ b/test/integration/full-content/fixtures/core__image.html
@@ -1,3 +1,3 @@
-
+
diff --git a/test/integration/full-content/fixtures/core__image.parsed.json b/test/integration/full-content/fixtures/core__image.parsed.json
index fff414d9b01ba..d461ce28ef22b 100644
--- a/test/integration/full-content/fixtures/core__image.parsed.json
+++ b/test/integration/full-content/fixtures/core__image.parsed.json
@@ -1,7 +1,10 @@
[
{
"blockName": "core/image",
- "attrs": {},
+ "attrs": {
+ "url": "https://cldup.com/uuUqE_dXzy.jpg",
+ "caption": ""
+ },
"innerBlocks": [],
"innerHTML": "\n
\n"
},
diff --git a/test/integration/full-content/fixtures/core__image.serialized.html b/test/integration/full-content/fixtures/core__image.serialized.html
index 5dfb0bac3e5b7..97bfce4a8d97f 100644
--- a/test/integration/full-content/fixtures/core__image.serialized.html
+++ b/test/integration/full-content/fixtures/core__image.serialized.html
@@ -1,3 +1,3 @@
-
+
diff --git a/test/integration/full-content/fixtures/core__image__attachment-link.html b/test/integration/full-content/fixtures/core__image__attachment-link.html
index 908250d8ca249..e464ce00e8d25 100644
--- a/test/integration/full-content/fixtures/core__image__attachment-link.html
+++ b/test/integration/full-content/fixtures/core__image__attachment-link.html
@@ -1,3 +1,3 @@
-
+
diff --git a/test/integration/full-content/fixtures/core__image__attachment-link.parsed.json b/test/integration/full-content/fixtures/core__image__attachment-link.parsed.json
index e5da07242d556..82403a176fda6 100644
--- a/test/integration/full-content/fixtures/core__image__attachment-link.parsed.json
+++ b/test/integration/full-content/fixtures/core__image__attachment-link.parsed.json
@@ -2,6 +2,9 @@
{
"blockName": "core/image",
"attrs": {
+ "url":"https://cldup.com/uuUqE_dXzy.jpg",
+ "caption":"",
+ "href":"http://localhost:8888/?attachment_id=7",
"linkDestination": "attachment"
},
"innerBlocks": [],
diff --git a/test/integration/full-content/fixtures/core__image__attachment-link.serialized.html b/test/integration/full-content/fixtures/core__image__attachment-link.serialized.html
index f5ebb9af4182c..15d9081562b65 100644
--- a/test/integration/full-content/fixtures/core__image__attachment-link.serialized.html
+++ b/test/integration/full-content/fixtures/core__image__attachment-link.serialized.html
@@ -1,3 +1,3 @@
-
+
diff --git a/test/integration/full-content/fixtures/core__image__center-caption.html b/test/integration/full-content/fixtures/core__image__center-caption.html
index bfe40d190fa66..21a83136d267b 100644
--- a/test/integration/full-content/fixtures/core__image__center-caption.html
+++ b/test/integration/full-content/fixtures/core__image__center-caption.html
@@ -1,3 +1,3 @@
-
+
Give it a try. Press the "really wide" button on the image toolbar.
diff --git a/test/integration/full-content/fixtures/core__image__center-caption.parsed.json b/test/integration/full-content/fixtures/core__image__center-caption.parsed.json
index 290057c2876a7..3e599fe75bafb 100644
--- a/test/integration/full-content/fixtures/core__image__center-caption.parsed.json
+++ b/test/integration/full-content/fixtures/core__image__center-caption.parsed.json
@@ -2,6 +2,8 @@
{
"blockName": "core/image",
"attrs": {
+ "url":"https://cldup.com/YLYhpou2oq.jpg",
+ "caption":"Give it a try. Press the \u0022really wide\u0022 button on the image toolbar.",
"align": "center"
},
"innerBlocks": [],
diff --git a/test/integration/full-content/fixtures/core__image__center-caption.serialized.html b/test/integration/full-content/fixtures/core__image__center-caption.serialized.html
index 3410b04fdf121..b9c8c2f1726e3 100644
--- a/test/integration/full-content/fixtures/core__image__center-caption.serialized.html
+++ b/test/integration/full-content/fixtures/core__image__center-caption.serialized.html
@@ -1,3 +1,3 @@
-
+
Give it a try. Press the "really wide" button on the image toolbar.
diff --git a/test/integration/full-content/fixtures/core__image__custom-link.html b/test/integration/full-content/fixtures/core__image__custom-link.html
index 353dc5376b7a4..0d488d07a8f09 100644
--- a/test/integration/full-content/fixtures/core__image__custom-link.html
+++ b/test/integration/full-content/fixtures/core__image__custom-link.html
@@ -1,3 +1,3 @@
-
+
diff --git a/test/integration/full-content/fixtures/core__image__custom-link.parsed.json b/test/integration/full-content/fixtures/core__image__custom-link.parsed.json
index d814737aaedf2..a8b6b996c0059 100644
--- a/test/integration/full-content/fixtures/core__image__custom-link.parsed.json
+++ b/test/integration/full-content/fixtures/core__image__custom-link.parsed.json
@@ -2,6 +2,9 @@
{
"blockName": "core/image",
"attrs": {
+ "url":"https://cldup.com/uuUqE_dXzy.jpg",
+ "caption":"",
+ "href":"https://wordpress.org/",
"linkDestination": "custom"
},
"innerBlocks": [],
diff --git a/test/integration/full-content/fixtures/core__image__custom-link.serialized.html b/test/integration/full-content/fixtures/core__image__custom-link.serialized.html
index 47357bea6b9d4..7618c8f3634c0 100644
--- a/test/integration/full-content/fixtures/core__image__custom-link.serialized.html
+++ b/test/integration/full-content/fixtures/core__image__custom-link.serialized.html
@@ -1,3 +1,3 @@
-
+
diff --git a/test/integration/full-content/fixtures/core__image__media-link.html b/test/integration/full-content/fixtures/core__image__media-link.html
index 90b3d227117b0..cd79ad5ec5efd 100644
--- a/test/integration/full-content/fixtures/core__image__media-link.html
+++ b/test/integration/full-content/fixtures/core__image__media-link.html
@@ -1,3 +1,3 @@
-
+
diff --git a/test/integration/full-content/fixtures/core__image__media-link.parsed.json b/test/integration/full-content/fixtures/core__image__media-link.parsed.json
index 345c5d4e258ed..328f27339ea2a 100644
--- a/test/integration/full-content/fixtures/core__image__media-link.parsed.json
+++ b/test/integration/full-content/fixtures/core__image__media-link.parsed.json
@@ -2,6 +2,9 @@
{
"blockName": "core/image",
"attrs": {
+ "url":"https://cldup.com/uuUqE_dXzy.jpg",
+ "caption":"",
+ "href":"https://cldup.com/uuUqE_dXzy.jpg",
"linkDestination": "media"
},
"innerBlocks": [],
diff --git a/test/integration/full-content/fixtures/core__image__media-link.serialized.html b/test/integration/full-content/fixtures/core__image__media-link.serialized.html
index 721abac903ff3..b414291532ed5 100644
--- a/test/integration/full-content/fixtures/core__image__media-link.serialized.html
+++ b/test/integration/full-content/fixtures/core__image__media-link.serialized.html
@@ -1,3 +1,3 @@
-
+