diff --git a/blocks/api/serializer.js b/blocks/api/serializer.js index 836afa5fc0db7..78b93d29535db 100644 --- a/blocks/api/serializer.js +++ b/blocks/api/serializer.js @@ -1,13 +1,14 @@ /** * External dependencies */ -import { isEmpty, map, reduce } from 'lodash'; +import { isEmpty, map, reduce, kebabCase, isObject } from 'lodash'; import { html as beautifyHtml } from 'js-beautify'; +import classnames from 'classnames'; /** * WordPress dependencies */ -import { Component, createElement, renderToString } from 'element'; +import { Component, createElement, renderToString, cloneElement, Children } from 'element'; /** * Internal dependencies @@ -16,14 +17,29 @@ import { getBlockType } from './registration'; import { parseBlockAttributes } from './parser'; /** - * Given a block's save render implementation and attributes, returns the + * Returns the block's default classname from its name + * + * @param {String} blockName The block name + * @return {string} The block's default class + */ +export function getBlockDefaultClassname( blockName ) { + // Drop the namespace "core/"" for core blocks only + const match = /^([a-z0-9-]+)\/([a-z0-9-]+)$/.exec( blockName ); + const sanitizedBlockName = match[ 1 ] === 'core' ? match[ 2 ] : blockName; + + return `wp-block-${ kebabCase( sanitizedBlockName ) }`; +} + +/** + * Given a block type containg a save render implementation and attributes, returns the * static markup to be saved. * - * @param {Function|WPComponent} save Save render implementation + * @param {Object} blockType Block type * @param {Object} attributes Block attributes * @return {string} Save content */ -export function getSaveContent( save, attributes ) { +export function getSaveContent( blockType, attributes ) { + const { save, className = getBlockDefaultClassname( blockType.name ) } = blockType; let rawContent; if ( save.prototype instanceof Component ) { @@ -37,8 +53,19 @@ export function getSaveContent( save, attributes ) { } } + // Adding a generic classname + const addClassnameToElement = ( element ) => { + if ( ! element || ! isObject( element ) || ! className ) { + return element; + } + + const updatedClassName = classnames( element.props.className, className ); + return cloneElement( element, { className: updatedClassName } ); + }; + const contentWithClassname = Children.map( rawContent, addClassnameToElement ); + // Otherwise, infer as element - return renderToString( rawContent ); + return renderToString( contentWithClassname ); } const escapeDoubleQuotes = value => value.replace( /"/g, '\"' ); @@ -107,7 +134,7 @@ function asNameValuePair( value, key ) { export function serializeBlock( block ) { const blockName = block.name; const blockType = getBlockType( blockName ); - const saveContent = getSaveContent( blockType.save, block.attributes ); + const saveContent = getSaveContent( blockType, block.attributes ); const saveAttributes = getCommentAttributes( block.attributes, parseBlockAttributes( saveContent, blockType ) ); const serializedAttributes = ! isEmpty( saveAttributes ) diff --git a/blocks/api/test/serializer.js b/blocks/api/test/serializer.js index 810cfd9ccc629..32b7ebba2eb11 100644 --- a/blocks/api/test/serializer.js +++ b/blocks/api/test/serializer.js @@ -25,7 +25,10 @@ describe( 'block serializer', () => { context( 'function save', () => { it( 'should return string verbatim', () => { const saved = getSaveContent( - ( { attributes } ) => attributes.fruit, + { + save: ( { attributes } ) => attributes.fruit, + name: 'core/fruit', + }, { fruit: 'Bananas' } ); @@ -34,7 +37,48 @@ describe( 'block serializer', () => { it( 'should return element as string if save returns element', () => { const saved = getSaveContent( - ( { attributes } ) => createElement( 'div', null, attributes.fruit ), + { + save: ( { attributes } ) => createElement( 'div', null, attributes.fruit ), + name: 'core/fruit', + }, + { fruit: 'Bananas' } + ); + + expect( saved ).to.equal( '
Ribs & Chicken
\n'; + const expectedPostContent = '\nRibs & Chicken
\n'; expect( serialize( blockList ) ).to.eql( expectedPostContent ); } ); diff --git a/blocks/library/heading/index.js b/blocks/library/heading/index.js index 05a432c667392..0619e13f071c0 100644 --- a/blocks/library/heading/index.js +++ b/blocks/library/heading/index.js @@ -26,6 +26,8 @@ registerBlockType( 'core/heading', { category: 'common', + className: false, + attributes: { content: children( 'h1,h2,h3,h4,h5,h6' ), nodeName: prop( 'h1,h2,h3,h4,h5,h6', 'nodeName' ), diff --git a/blocks/library/html/index.js b/blocks/library/html/index.js index bc5c05b4a84cd..554781c834cad 100644 --- a/blocks/library/html/index.js +++ b/blocks/library/html/index.js @@ -25,6 +25,8 @@ registerBlockType( 'core/html', { category: 'formatting', + className: false, + attributes: { content: children(), }, diff --git a/blocks/library/list/index.js b/blocks/library/list/index.js index 750cce361564a..a31d69daf4db2 100644 --- a/blocks/library/list/index.js +++ b/blocks/library/list/index.js @@ -74,6 +74,8 @@ registerBlockType( 'core/list', { values: children( 'ol,ul' ), }, + className: false, + transforms: { from: [ { diff --git a/blocks/library/text/index.js b/blocks/library/text/index.js index f4663ec8f34fe..4afc842abc602 100644 --- a/blocks/library/text/index.js +++ b/blocks/library/text/index.js @@ -23,6 +23,8 @@ registerBlockType( 'core/text', { category: 'common', + className: false, + attributes: { content: query( 'p', children() ), }, diff --git a/blocks/test/fixtures/core-button-center.html b/blocks/test/fixtures/core-button-center.html index b80f97864c293..9e1899a30fec2 100644 --- a/blocks/test/fixtures/core-button-center.html +++ b/blocks/test/fixtures/core-button-center.html @@ -1,3 +1,3 @@ - + diff --git a/blocks/test/fixtures/core-button-center.serialized.html b/blocks/test/fixtures/core-button-center.serialized.html index c360f9e247a91..d86f39e98c299 100644 --- a/blocks/test/fixtures/core-button-center.serialized.html +++ b/blocks/test/fixtures/core-button-center.serialized.html @@ -1,4 +1,4 @@ - + diff --git a/blocks/test/fixtures/core-code.html b/blocks/test/fixtures/core-code.html index 0c00ab494b2f9..5d3f951c17f71 100644 --- a/blocks/test/fixtures/core-code.html +++ b/blocks/test/fixtures/core-code.html @@ -1,5 +1,5 @@ -export default function MyButton() {
+export default function MyButton() {
return <Button>Click Me!</Button>;
}
diff --git a/blocks/test/fixtures/core-code.serialized.html b/blocks/test/fixtures/core-code.serialized.html
index fbf78a33703c0..695395457a7c9 100644
--- a/blocks/test/fixtures/core-code.serialized.html
+++ b/blocks/test/fixtures/core-code.serialized.html
@@ -1,5 +1,5 @@
-export default function MyButton() {
+export default function MyButton() {
return <Button>Click Me!</Button>;
}
diff --git a/blocks/test/fixtures/core-cover-image.serialized.html b/blocks/test/fixtures/core-cover-image.serialized.html
index 7e8bcbb7014ad..c4858e6d55089 100644
--- a/blocks/test/fixtures/core-cover-image.serialized.html
+++ b/blocks/test/fixtures/core-cover-image.serialized.html
@@ -1,5 +1,5 @@
-
+
Guten Berg!
diff --git a/blocks/test/fixtures/core-embed.html b/blocks/test/fixtures/core-embed.html
index af8b876de71e0..4a3a026dbbfaa 100644
--- a/blocks/test/fixtures/core-embed.html
+++ b/blocks/test/fixtures/core-embed.html
@@ -1,5 +1,5 @@
-
+
https://example.com/
Embedded content from an example URL
diff --git a/blocks/test/fixtures/core-embed.serialized.html b/blocks/test/fixtures/core-embed.serialized.html
index b8b1e50e7afef..3a48b93509de9 100644
--- a/blocks/test/fixtures/core-embed.serialized.html
+++ b/blocks/test/fixtures/core-embed.serialized.html
@@ -1,5 +1,5 @@
-
+
https://example.com/
Embedded content from an example URL
diff --git a/blocks/test/fixtures/core-embedanimoto.html b/blocks/test/fixtures/core-embedanimoto.html
index 0e464a377f248..0b636c81362b2 100644
--- a/blocks/test/fixtures/core-embedanimoto.html
+++ b/blocks/test/fixtures/core-embedanimoto.html
@@ -1,5 +1,5 @@
-
+
https://animoto.com/
Embedded content from animoto
diff --git a/blocks/test/fixtures/core-embedanimoto.serialized.html b/blocks/test/fixtures/core-embedanimoto.serialized.html
index e33c67b8256d6..a007c6b7bedad 100644
--- a/blocks/test/fixtures/core-embedanimoto.serialized.html
+++ b/blocks/test/fixtures/core-embedanimoto.serialized.html
@@ -1,5 +1,5 @@
-
+
https://animoto.com/
Embedded content from animoto
diff --git a/blocks/test/fixtures/core-embedcloudup.html b/blocks/test/fixtures/core-embedcloudup.html
index 4650c84b4b703..4a9e1505aab67 100644
--- a/blocks/test/fixtures/core-embedcloudup.html
+++ b/blocks/test/fixtures/core-embedcloudup.html
@@ -1,5 +1,5 @@
-
+
https://cloudup.com/
Embedded content from cloudup
diff --git a/blocks/test/fixtures/core-embedcloudup.serialized.html b/blocks/test/fixtures/core-embedcloudup.serialized.html
index 02951c1171ec5..fda04d9fc6808 100644
--- a/blocks/test/fixtures/core-embedcloudup.serialized.html
+++ b/blocks/test/fixtures/core-embedcloudup.serialized.html
@@ -1,5 +1,5 @@
-
+
https://cloudup.com/
Embedded content from cloudup
diff --git a/blocks/test/fixtures/core-embedcollegehumor.html b/blocks/test/fixtures/core-embedcollegehumor.html
index 5ecdbc3e4dd88..7cc4683fbd3b6 100644
--- a/blocks/test/fixtures/core-embedcollegehumor.html
+++ b/blocks/test/fixtures/core-embedcollegehumor.html
@@ -1,5 +1,5 @@
-
+
https://collegehumor.com/
Embedded content from collegehumor
diff --git a/blocks/test/fixtures/core-embedcollegehumor.serialized.html b/blocks/test/fixtures/core-embedcollegehumor.serialized.html
index 82ffd18b7c829..71317f6a8b038 100644
--- a/blocks/test/fixtures/core-embedcollegehumor.serialized.html
+++ b/blocks/test/fixtures/core-embedcollegehumor.serialized.html
@@ -1,5 +1,5 @@
-
+
https://collegehumor.com/
Embedded content from collegehumor
diff --git a/blocks/test/fixtures/core-embeddailymotion.html b/blocks/test/fixtures/core-embeddailymotion.html
index 08bed7270fe70..3d5e11f2c589d 100644
--- a/blocks/test/fixtures/core-embeddailymotion.html
+++ b/blocks/test/fixtures/core-embeddailymotion.html
@@ -1,5 +1,5 @@
-
+
https://dailymotion.com/
Embedded content from dailymotion
diff --git a/blocks/test/fixtures/core-embeddailymotion.serialized.html b/blocks/test/fixtures/core-embeddailymotion.serialized.html
index 62f55871d1e1f..255ddf1b0dce2 100644
--- a/blocks/test/fixtures/core-embeddailymotion.serialized.html
+++ b/blocks/test/fixtures/core-embeddailymotion.serialized.html
@@ -1,5 +1,5 @@
-
+
https://dailymotion.com/
Embedded content from dailymotion
diff --git a/blocks/test/fixtures/core-embedfacebook.html b/blocks/test/fixtures/core-embedfacebook.html
index 4a5044c13d6cd..676639abeabe7 100644
--- a/blocks/test/fixtures/core-embedfacebook.html
+++ b/blocks/test/fixtures/core-embedfacebook.html
@@ -1,5 +1,5 @@
-
+
https://facebook.com/
Embedded content from facebook
diff --git a/blocks/test/fixtures/core-embedfacebook.serialized.html b/blocks/test/fixtures/core-embedfacebook.serialized.html
index efda5813641da..32f7c6f2933c2 100644
--- a/blocks/test/fixtures/core-embedfacebook.serialized.html
+++ b/blocks/test/fixtures/core-embedfacebook.serialized.html
@@ -1,5 +1,5 @@
-
+
https://facebook.com/
Embedded content from facebook
diff --git a/blocks/test/fixtures/core-embedflickr.html b/blocks/test/fixtures/core-embedflickr.html
index 2325790efcab2..e3b40b68cfb11 100644
--- a/blocks/test/fixtures/core-embedflickr.html
+++ b/blocks/test/fixtures/core-embedflickr.html
@@ -1,5 +1,5 @@
-
+
https://flickr.com/
Embedded content from flickr
diff --git a/blocks/test/fixtures/core-embedflickr.serialized.html b/blocks/test/fixtures/core-embedflickr.serialized.html
index 4d86c30f5b881..8253ec7d974ee 100644
--- a/blocks/test/fixtures/core-embedflickr.serialized.html
+++ b/blocks/test/fixtures/core-embedflickr.serialized.html
@@ -1,5 +1,5 @@
-
+
https://flickr.com/
Embedded content from flickr
diff --git a/blocks/test/fixtures/core-embedfunnyordie.html b/blocks/test/fixtures/core-embedfunnyordie.html
index 67e845a743e95..d4ceb296ebeb0 100644
--- a/blocks/test/fixtures/core-embedfunnyordie.html
+++ b/blocks/test/fixtures/core-embedfunnyordie.html
@@ -1,5 +1,5 @@
-
+
https://funnyordie.com/
Embedded content from funnyordie
diff --git a/blocks/test/fixtures/core-embedfunnyordie.serialized.html b/blocks/test/fixtures/core-embedfunnyordie.serialized.html
index bba227b1c1956..a287abe114dbc 100644
--- a/blocks/test/fixtures/core-embedfunnyordie.serialized.html
+++ b/blocks/test/fixtures/core-embedfunnyordie.serialized.html
@@ -1,5 +1,5 @@
-
+
https://funnyordie.com/
Embedded content from funnyordie
diff --git a/blocks/test/fixtures/core-embedhulu.html b/blocks/test/fixtures/core-embedhulu.html
index 749b3ecb99aee..461ccafc651f9 100644
--- a/blocks/test/fixtures/core-embedhulu.html
+++ b/blocks/test/fixtures/core-embedhulu.html
@@ -1,5 +1,5 @@
-
+
https://hulu.com/
Embedded content from hulu
diff --git a/blocks/test/fixtures/core-embedhulu.serialized.html b/blocks/test/fixtures/core-embedhulu.serialized.html
index c9c5b31537a69..7bd8f13f5c54c 100644
--- a/blocks/test/fixtures/core-embedhulu.serialized.html
+++ b/blocks/test/fixtures/core-embedhulu.serialized.html
@@ -1,5 +1,5 @@
-
+
https://hulu.com/
Embedded content from hulu
diff --git a/blocks/test/fixtures/core-embedimgur.html b/blocks/test/fixtures/core-embedimgur.html
index 93f03e68d48d8..c99b387d89df5 100644
--- a/blocks/test/fixtures/core-embedimgur.html
+++ b/blocks/test/fixtures/core-embedimgur.html
@@ -1,5 +1,5 @@
-
+
https://imgur.com/
Embedded content from imgur
diff --git a/blocks/test/fixtures/core-embedimgur.serialized.html b/blocks/test/fixtures/core-embedimgur.serialized.html
index e1e3510c97eab..6c73894b42afa 100644
--- a/blocks/test/fixtures/core-embedimgur.serialized.html
+++ b/blocks/test/fixtures/core-embedimgur.serialized.html
@@ -1,5 +1,5 @@
-
+
https://imgur.com/
Embedded content from imgur
diff --git a/blocks/test/fixtures/core-embedinstagram.html b/blocks/test/fixtures/core-embedinstagram.html
index 12dc0d1ec0853..5532e1efac3d7 100644
--- a/blocks/test/fixtures/core-embedinstagram.html
+++ b/blocks/test/fixtures/core-embedinstagram.html
@@ -1,5 +1,5 @@
-
+
https://instagram.com/
Embedded content from instagram
diff --git a/blocks/test/fixtures/core-embedinstagram.serialized.html b/blocks/test/fixtures/core-embedinstagram.serialized.html
index 018a38ab8d52b..da883aaa69f8d 100644
--- a/blocks/test/fixtures/core-embedinstagram.serialized.html
+++ b/blocks/test/fixtures/core-embedinstagram.serialized.html
@@ -1,5 +1,5 @@
-
+
https://instagram.com/
Embedded content from instagram
diff --git a/blocks/test/fixtures/core-embedissuu.html b/blocks/test/fixtures/core-embedissuu.html
index 6849278719bc4..d7b113d69ebea 100644
--- a/blocks/test/fixtures/core-embedissuu.html
+++ b/blocks/test/fixtures/core-embedissuu.html
@@ -1,5 +1,5 @@
-
+
https://issuu.com/
Embedded content from issuu
diff --git a/blocks/test/fixtures/core-embedissuu.serialized.html b/blocks/test/fixtures/core-embedissuu.serialized.html
index 18903978bd6e1..523979b284dda 100644
--- a/blocks/test/fixtures/core-embedissuu.serialized.html
+++ b/blocks/test/fixtures/core-embedissuu.serialized.html
@@ -1,5 +1,5 @@
-
+
https://issuu.com/
Embedded content from issuu
diff --git a/blocks/test/fixtures/core-embedkickstarter.html b/blocks/test/fixtures/core-embedkickstarter.html
index dbd11a91fe39b..9c0ab3683e23e 100644
--- a/blocks/test/fixtures/core-embedkickstarter.html
+++ b/blocks/test/fixtures/core-embedkickstarter.html
@@ -1,5 +1,5 @@
-
+
https://kickstarter.com/
Embedded content from kickstarter
diff --git a/blocks/test/fixtures/core-embedkickstarter.serialized.html b/blocks/test/fixtures/core-embedkickstarter.serialized.html
index 600c019a80342..3cfeeaf23c71d 100644
--- a/blocks/test/fixtures/core-embedkickstarter.serialized.html
+++ b/blocks/test/fixtures/core-embedkickstarter.serialized.html
@@ -1,5 +1,5 @@
-
+
https://kickstarter.com/
Embedded content from kickstarter
diff --git a/blocks/test/fixtures/core-embedmeetupcom.html b/blocks/test/fixtures/core-embedmeetupcom.html
index f72f0acfcea7e..1b85b5ec15102 100644
--- a/blocks/test/fixtures/core-embedmeetupcom.html
+++ b/blocks/test/fixtures/core-embedmeetupcom.html
@@ -1,5 +1,5 @@
-
+
https://meetupcom.com/
Embedded content from meetupcom
diff --git a/blocks/test/fixtures/core-embedmeetupcom.serialized.html b/blocks/test/fixtures/core-embedmeetupcom.serialized.html
index c271a0550b38c..f9482afceec40 100644
--- a/blocks/test/fixtures/core-embedmeetupcom.serialized.html
+++ b/blocks/test/fixtures/core-embedmeetupcom.serialized.html
@@ -1,5 +1,5 @@
-
+
https://meetupcom.com/
Embedded content from meetupcom
diff --git a/blocks/test/fixtures/core-embedmixcloud.html b/blocks/test/fixtures/core-embedmixcloud.html
index bf79ac8537924..694bf7d7198ae 100644
--- a/blocks/test/fixtures/core-embedmixcloud.html
+++ b/blocks/test/fixtures/core-embedmixcloud.html
@@ -1,5 +1,5 @@
-
+
https://mixcloud.com/
Embedded content from mixcloud
diff --git a/blocks/test/fixtures/core-embedmixcloud.serialized.html b/blocks/test/fixtures/core-embedmixcloud.serialized.html
index dd53c339d93a0..553aaeedaf730 100644
--- a/blocks/test/fixtures/core-embedmixcloud.serialized.html
+++ b/blocks/test/fixtures/core-embedmixcloud.serialized.html
@@ -1,5 +1,5 @@
-
+
https://mixcloud.com/
Embedded content from mixcloud
diff --git a/blocks/test/fixtures/core-embedphotobucket.html b/blocks/test/fixtures/core-embedphotobucket.html
index ba8170950c372..c6a485ac6697f 100644
--- a/blocks/test/fixtures/core-embedphotobucket.html
+++ b/blocks/test/fixtures/core-embedphotobucket.html
@@ -1,5 +1,5 @@
-
+
https://photobucket.com/
Embedded content from photobucket
diff --git a/blocks/test/fixtures/core-embedphotobucket.serialized.html b/blocks/test/fixtures/core-embedphotobucket.serialized.html
index e2631ab04ceee..c6ab742e7acb9 100644
--- a/blocks/test/fixtures/core-embedphotobucket.serialized.html
+++ b/blocks/test/fixtures/core-embedphotobucket.serialized.html
@@ -1,5 +1,5 @@
-
+
https://photobucket.com/
Embedded content from photobucket
diff --git a/blocks/test/fixtures/core-embedpolldaddy.html b/blocks/test/fixtures/core-embedpolldaddy.html
index 4176efd62628d..2100a77678f8b 100644
--- a/blocks/test/fixtures/core-embedpolldaddy.html
+++ b/blocks/test/fixtures/core-embedpolldaddy.html
@@ -1,5 +1,5 @@
-
+
https://polldaddy.com/
Embedded content from polldaddy
diff --git a/blocks/test/fixtures/core-embedpolldaddy.serialized.html b/blocks/test/fixtures/core-embedpolldaddy.serialized.html
index 20f6eba0732f3..eb03ce80833c7 100644
--- a/blocks/test/fixtures/core-embedpolldaddy.serialized.html
+++ b/blocks/test/fixtures/core-embedpolldaddy.serialized.html
@@ -1,5 +1,5 @@
-
+
https://polldaddy.com/
Embedded content from polldaddy
diff --git a/blocks/test/fixtures/core-embedreddit.html b/blocks/test/fixtures/core-embedreddit.html
index 3537909ad8430..e65afcbd57a50 100644
--- a/blocks/test/fixtures/core-embedreddit.html
+++ b/blocks/test/fixtures/core-embedreddit.html
@@ -1,5 +1,5 @@
-
+
https://reddit.com/
Embedded content from reddit
diff --git a/blocks/test/fixtures/core-embedreddit.serialized.html b/blocks/test/fixtures/core-embedreddit.serialized.html
index 8fda9380fb209..132abb68d19bf 100644
--- a/blocks/test/fixtures/core-embedreddit.serialized.html
+++ b/blocks/test/fixtures/core-embedreddit.serialized.html
@@ -1,5 +1,5 @@
-
+
https://reddit.com/
Embedded content from reddit
diff --git a/blocks/test/fixtures/core-embedreverbnation.html b/blocks/test/fixtures/core-embedreverbnation.html
index 3e03c3404f757..5cbaf057fc287 100644
--- a/blocks/test/fixtures/core-embedreverbnation.html
+++ b/blocks/test/fixtures/core-embedreverbnation.html
@@ -1,5 +1,5 @@
-
+
https://reverbnation.com/
Embedded content from reverbnation
diff --git a/blocks/test/fixtures/core-embedreverbnation.serialized.html b/blocks/test/fixtures/core-embedreverbnation.serialized.html
index 3f5686dad96cf..423d043a84844 100644
--- a/blocks/test/fixtures/core-embedreverbnation.serialized.html
+++ b/blocks/test/fixtures/core-embedreverbnation.serialized.html
@@ -1,5 +1,5 @@
-
+
https://reverbnation.com/
Embedded content from reverbnation
diff --git a/blocks/test/fixtures/core-embedscreencast.html b/blocks/test/fixtures/core-embedscreencast.html
index 75e7990c480f7..13a6bfc932442 100644
--- a/blocks/test/fixtures/core-embedscreencast.html
+++ b/blocks/test/fixtures/core-embedscreencast.html
@@ -1,5 +1,5 @@
-
+
https://screencast.com/
Embedded content from screencast
diff --git a/blocks/test/fixtures/core-embedscreencast.serialized.html b/blocks/test/fixtures/core-embedscreencast.serialized.html
index 2d6b546863769..531f502cbf2d2 100644
--- a/blocks/test/fixtures/core-embedscreencast.serialized.html
+++ b/blocks/test/fixtures/core-embedscreencast.serialized.html
@@ -1,5 +1,5 @@
-
+
https://screencast.com/
Embedded content from screencast
diff --git a/blocks/test/fixtures/core-embedscribd.html b/blocks/test/fixtures/core-embedscribd.html
index 4971e8c943842..c1fa083daa8b7 100644
--- a/blocks/test/fixtures/core-embedscribd.html
+++ b/blocks/test/fixtures/core-embedscribd.html
@@ -1,5 +1,5 @@
-
+
https://scribd.com/
Embedded content from scribd
diff --git a/blocks/test/fixtures/core-embedscribd.serialized.html b/blocks/test/fixtures/core-embedscribd.serialized.html
index 06069125228bd..297a990fbfc76 100644
--- a/blocks/test/fixtures/core-embedscribd.serialized.html
+++ b/blocks/test/fixtures/core-embedscribd.serialized.html
@@ -1,5 +1,5 @@
-
+
https://scribd.com/
Embedded content from scribd
diff --git a/blocks/test/fixtures/core-embedslideshare.html b/blocks/test/fixtures/core-embedslideshare.html
index abd33acdf7c46..22122bfbc7fbc 100644
--- a/blocks/test/fixtures/core-embedslideshare.html
+++ b/blocks/test/fixtures/core-embedslideshare.html
@@ -1,5 +1,5 @@
-
+
https://slideshare.com/
Embedded content from slideshare
diff --git a/blocks/test/fixtures/core-embedslideshare.serialized.html b/blocks/test/fixtures/core-embedslideshare.serialized.html
index 8184fc98e5a1a..b36aa00eae92e 100644
--- a/blocks/test/fixtures/core-embedslideshare.serialized.html
+++ b/blocks/test/fixtures/core-embedslideshare.serialized.html
@@ -1,5 +1,5 @@
-
+
https://slideshare.com/
Embedded content from slideshare
diff --git a/blocks/test/fixtures/core-embedsmugmug.html b/blocks/test/fixtures/core-embedsmugmug.html
index 7dc99c1cb7dfb..051ac4f2a5dfe 100644
--- a/blocks/test/fixtures/core-embedsmugmug.html
+++ b/blocks/test/fixtures/core-embedsmugmug.html
@@ -1,5 +1,5 @@
-
+
https://smugmug.com/
Embedded content from smugmug
diff --git a/blocks/test/fixtures/core-embedsmugmug.serialized.html b/blocks/test/fixtures/core-embedsmugmug.serialized.html
index 4755f4de1a2c5..861ea30e9cd8a 100644
--- a/blocks/test/fixtures/core-embedsmugmug.serialized.html
+++ b/blocks/test/fixtures/core-embedsmugmug.serialized.html
@@ -1,5 +1,5 @@
-
+
https://smugmug.com/
Embedded content from smugmug
diff --git a/blocks/test/fixtures/core-embedsoundcloud.html b/blocks/test/fixtures/core-embedsoundcloud.html
index c02c19b249a0b..71861f3fc70f3 100644
--- a/blocks/test/fixtures/core-embedsoundcloud.html
+++ b/blocks/test/fixtures/core-embedsoundcloud.html
@@ -1,5 +1,5 @@
-
+
https://soundcloud.com/
Embedded content from soundcloud
diff --git a/blocks/test/fixtures/core-embedsoundcloud.serialized.html b/blocks/test/fixtures/core-embedsoundcloud.serialized.html
index 70688f2cad848..737adfdf9783e 100644
--- a/blocks/test/fixtures/core-embedsoundcloud.serialized.html
+++ b/blocks/test/fixtures/core-embedsoundcloud.serialized.html
@@ -1,5 +1,5 @@
-
+
https://soundcloud.com/
Embedded content from soundcloud
diff --git a/blocks/test/fixtures/core-embedspeaker.html b/blocks/test/fixtures/core-embedspeaker.html
index a7a9332fff5d2..b867ca3306628 100644
--- a/blocks/test/fixtures/core-embedspeaker.html
+++ b/blocks/test/fixtures/core-embedspeaker.html
@@ -1,5 +1,5 @@
-
+
https://speaker.com/
Embedded content from speaker
diff --git a/blocks/test/fixtures/core-embedspeaker.serialized.html b/blocks/test/fixtures/core-embedspeaker.serialized.html
index 77b6efdd62940..6ff3f887897f4 100644
--- a/blocks/test/fixtures/core-embedspeaker.serialized.html
+++ b/blocks/test/fixtures/core-embedspeaker.serialized.html
@@ -1,5 +1,5 @@
-
+
https://speaker.com/
Embedded content from speaker
diff --git a/blocks/test/fixtures/core-embedspotify.html b/blocks/test/fixtures/core-embedspotify.html
index bfe67cb9034fc..c7d30a15cce87 100644
--- a/blocks/test/fixtures/core-embedspotify.html
+++ b/blocks/test/fixtures/core-embedspotify.html
@@ -1,5 +1,5 @@
-
+
https://spotify.com/
Embedded content from spotify
diff --git a/blocks/test/fixtures/core-embedspotify.serialized.html b/blocks/test/fixtures/core-embedspotify.serialized.html
index 8e88da9f4e3a2..76758f39e77f8 100644
--- a/blocks/test/fixtures/core-embedspotify.serialized.html
+++ b/blocks/test/fixtures/core-embedspotify.serialized.html
@@ -1,5 +1,5 @@
-
+
https://spotify.com/
Embedded content from spotify
diff --git a/blocks/test/fixtures/core-embedted.html b/blocks/test/fixtures/core-embedted.html
index eb58f0c2c3949..d2f5b32252003 100644
--- a/blocks/test/fixtures/core-embedted.html
+++ b/blocks/test/fixtures/core-embedted.html
@@ -1,5 +1,5 @@
-
+
https://ted.com/
Embedded content from ted
diff --git a/blocks/test/fixtures/core-embedted.serialized.html b/blocks/test/fixtures/core-embedted.serialized.html
index 5a9d51536f5da..d31e228747781 100644
--- a/blocks/test/fixtures/core-embedted.serialized.html
+++ b/blocks/test/fixtures/core-embedted.serialized.html
@@ -1,5 +1,5 @@
-
+
https://ted.com/
Embedded content from ted
diff --git a/blocks/test/fixtures/core-embedtumblr.html b/blocks/test/fixtures/core-embedtumblr.html
index ee07d48e887ba..5b61b112a44e4 100644
--- a/blocks/test/fixtures/core-embedtumblr.html
+++ b/blocks/test/fixtures/core-embedtumblr.html
@@ -1,5 +1,5 @@
-
+
https://tumblr.com/
Embedded content from tumblr
diff --git a/blocks/test/fixtures/core-embedtumblr.serialized.html b/blocks/test/fixtures/core-embedtumblr.serialized.html
index 8f1da3fb2e4d9..2143be60542c5 100644
--- a/blocks/test/fixtures/core-embedtumblr.serialized.html
+++ b/blocks/test/fixtures/core-embedtumblr.serialized.html
@@ -1,5 +1,5 @@
-
+
https://tumblr.com/
Embedded content from tumblr
diff --git a/blocks/test/fixtures/core-embedtwitter.html b/blocks/test/fixtures/core-embedtwitter.html
index 807a6bfd27604..b72be15a1f079 100644
--- a/blocks/test/fixtures/core-embedtwitter.html
+++ b/blocks/test/fixtures/core-embedtwitter.html
@@ -1,5 +1,5 @@
-
+
https://twitter.com/automattic
We are Automattic
diff --git a/blocks/test/fixtures/core-embedtwitter.serialized.html b/blocks/test/fixtures/core-embedtwitter.serialized.html
index bc214e661e01e..ae9ec684986a5 100644
--- a/blocks/test/fixtures/core-embedtwitter.serialized.html
+++ b/blocks/test/fixtures/core-embedtwitter.serialized.html
@@ -1,5 +1,5 @@
-
+
https://twitter.com/automattic
We are Automattic
diff --git a/blocks/test/fixtures/core-embedvideopress.html b/blocks/test/fixtures/core-embedvideopress.html
index ba2ff6bdc2e51..3169a6c898123 100644
--- a/blocks/test/fixtures/core-embedvideopress.html
+++ b/blocks/test/fixtures/core-embedvideopress.html
@@ -1,5 +1,5 @@
-
+
https://videopress.com/
Embedded content from videopress
diff --git a/blocks/test/fixtures/core-embedvideopress.serialized.html b/blocks/test/fixtures/core-embedvideopress.serialized.html
index 571972d53ff4d..f7f47f95158b0 100644
--- a/blocks/test/fixtures/core-embedvideopress.serialized.html
+++ b/blocks/test/fixtures/core-embedvideopress.serialized.html
@@ -1,5 +1,5 @@
-
+
https://videopress.com/
Embedded content from videopress
diff --git a/blocks/test/fixtures/core-embedvimeo.html b/blocks/test/fixtures/core-embedvimeo.html
index 81b4698f749c2..19a10852c9e5f 100644
--- a/blocks/test/fixtures/core-embedvimeo.html
+++ b/blocks/test/fixtures/core-embedvimeo.html
@@ -1,5 +1,5 @@
-
+
https://vimeo.com/
Embedded content from vimeo
diff --git a/blocks/test/fixtures/core-embedvimeo.serialized.html b/blocks/test/fixtures/core-embedvimeo.serialized.html
index eb1522a937aea..d822d6ea7842a 100644
--- a/blocks/test/fixtures/core-embedvimeo.serialized.html
+++ b/blocks/test/fixtures/core-embedvimeo.serialized.html
@@ -1,5 +1,5 @@
-
+
https://vimeo.com/
Embedded content from vimeo
diff --git a/blocks/test/fixtures/core-embedvine.html b/blocks/test/fixtures/core-embedvine.html
index b70558a5fc77b..cc59f6594119e 100644
--- a/blocks/test/fixtures/core-embedvine.html
+++ b/blocks/test/fixtures/core-embedvine.html
@@ -1,5 +1,5 @@
-
+
https://vine.com/
Embedded content from vine
diff --git a/blocks/test/fixtures/core-embedvine.serialized.html b/blocks/test/fixtures/core-embedvine.serialized.html
index df9496fb94081..5f16f77d73f44 100644
--- a/blocks/test/fixtures/core-embedvine.serialized.html
+++ b/blocks/test/fixtures/core-embedvine.serialized.html
@@ -1,5 +1,5 @@
-
+
https://vine.com/
Embedded content from vine
diff --git a/blocks/test/fixtures/core-embedwordpress.html b/blocks/test/fixtures/core-embedwordpress.html
index accd979b51f83..2d33a2f8bca5c 100644
--- a/blocks/test/fixtures/core-embedwordpress.html
+++ b/blocks/test/fixtures/core-embedwordpress.html
@@ -1,5 +1,5 @@
-
+
https://wordpress.com/
Embedded content from WordPress
diff --git a/blocks/test/fixtures/core-embedwordpress.serialized.html b/blocks/test/fixtures/core-embedwordpress.serialized.html
index 0905c1b166b45..6fb0d81613dfe 100644
--- a/blocks/test/fixtures/core-embedwordpress.serialized.html
+++ b/blocks/test/fixtures/core-embedwordpress.serialized.html
@@ -1,5 +1,5 @@
-
+
https://wordpress.com/
Embedded content from WordPress
diff --git a/blocks/test/fixtures/core-embedwordpresstv.html b/blocks/test/fixtures/core-embedwordpresstv.html
index eca3877f5ced8..f2639556cca0f 100644
--- a/blocks/test/fixtures/core-embedwordpresstv.html
+++ b/blocks/test/fixtures/core-embedwordpresstv.html
@@ -1,5 +1,5 @@
-
+
https://wordpresstv.com/
Embedded content from wordpresstv
diff --git a/blocks/test/fixtures/core-embedwordpresstv.serialized.html b/blocks/test/fixtures/core-embedwordpresstv.serialized.html
index bc6c773f16293..ce9ce84fc9a26 100644
--- a/blocks/test/fixtures/core-embedwordpresstv.serialized.html
+++ b/blocks/test/fixtures/core-embedwordpresstv.serialized.html
@@ -1,5 +1,5 @@
-
+
https://wordpresstv.com/
Embedded content from wordpresstv
diff --git a/blocks/test/fixtures/core-embedyoutube.html b/blocks/test/fixtures/core-embedyoutube.html
index e0832c00589d2..026763327b62a 100644
--- a/blocks/test/fixtures/core-embedyoutube.html
+++ b/blocks/test/fixtures/core-embedyoutube.html
@@ -1,5 +1,5 @@
-
+
https://youtube.com/
Embedded content from youtube
diff --git a/blocks/test/fixtures/core-embedyoutube.serialized.html b/blocks/test/fixtures/core-embedyoutube.serialized.html
index 02fa086d489e7..8c1a1265bf504 100644
--- a/blocks/test/fixtures/core-embedyoutube.serialized.html
+++ b/blocks/test/fixtures/core-embedyoutube.serialized.html
@@ -1,5 +1,5 @@
-
+
https://youtube.com/
Embedded content from youtube
diff --git a/blocks/test/fixtures/core-freeform.serialized.html b/blocks/test/fixtures/core-freeform.serialized.html
index d30114895dd4c..a31ee92b0385e 100644
--- a/blocks/test/fixtures/core-freeform.serialized.html
+++ b/blocks/test/fixtures/core-freeform.serialized.html
@@ -1,6 +1,6 @@
Testing freeform block with some
-
+
HTML content
diff --git a/blocks/test/fixtures/core-gallery.html b/blocks/test/fixtures/core-gallery.html
index 523a6732dd959..f60cc60da9abe 100644
--- a/blocks/test/fixtures/core-gallery.html
+++ b/blocks/test/fixtures/core-gallery.html
@@ -1,5 +1,5 @@
-
+
diff --git a/blocks/test/fixtures/core-gallery.serialized.html b/blocks/test/fixtures/core-gallery.serialized.html
index 09f55993d4fd9..4295680cc60b1 100644
--- a/blocks/test/fixtures/core-gallery.serialized.html
+++ b/blocks/test/fixtures/core-gallery.serialized.html
@@ -1,5 +1,5 @@
-
+
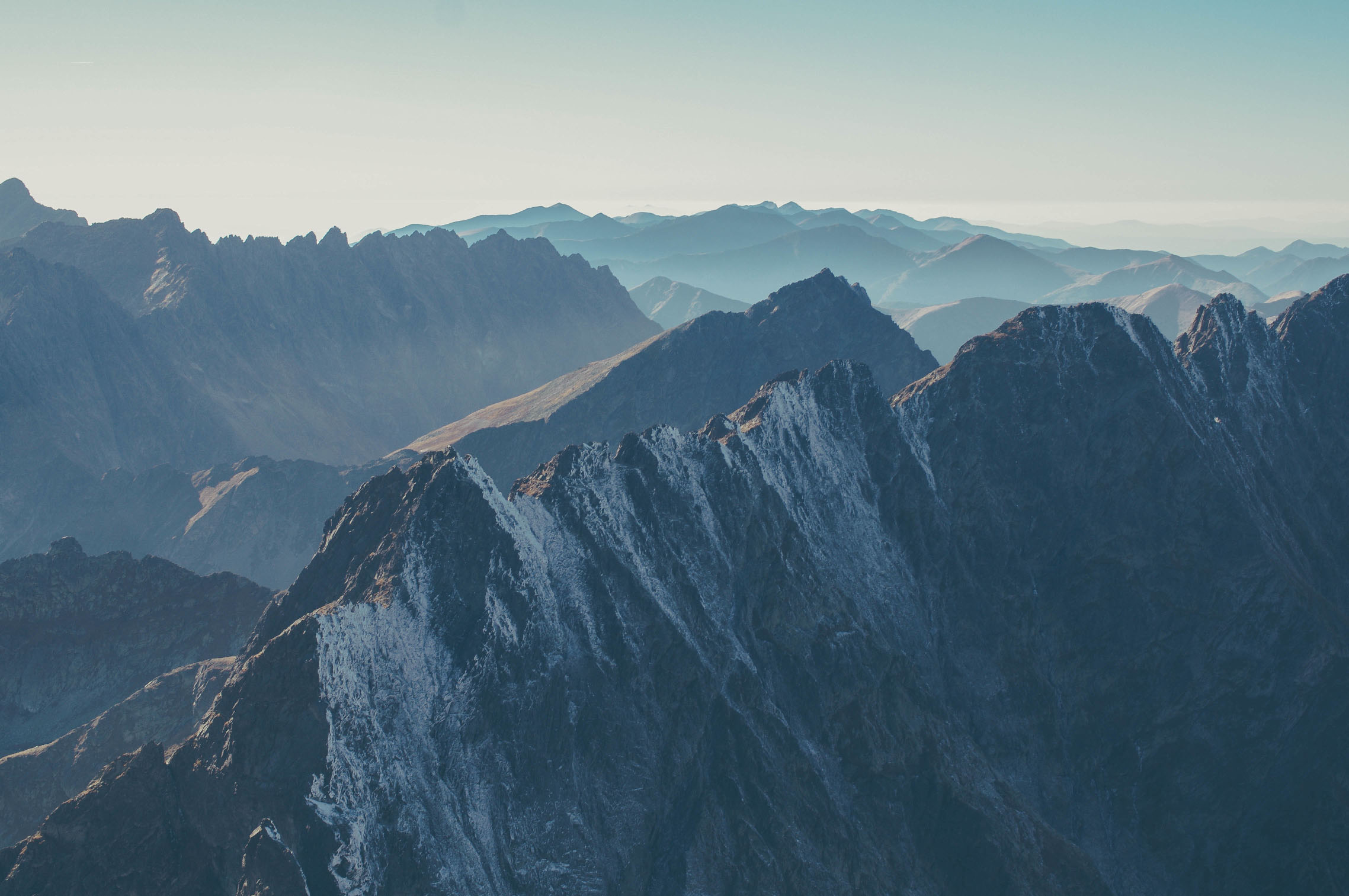

diff --git a/blocks/test/fixtures/core-image-center-caption.html b/blocks/test/fixtures/core-image-center-caption.html
index a528bacf46745..0aa89dced8561 100644
--- a/blocks/test/fixtures/core-image-center-caption.html
+++ b/blocks/test/fixtures/core-image-center-caption.html
@@ -1,3 +1,3 @@
-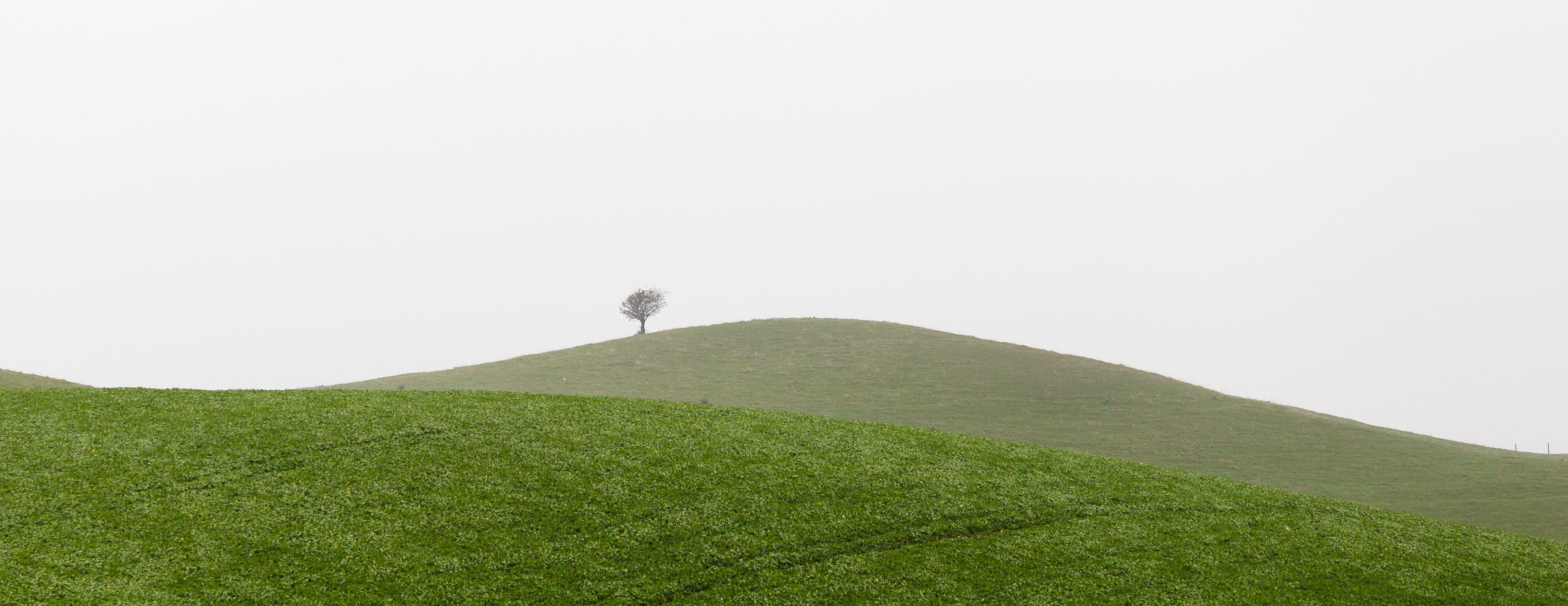
Give it a try. Press the "really wide" button on the image toolbar.
+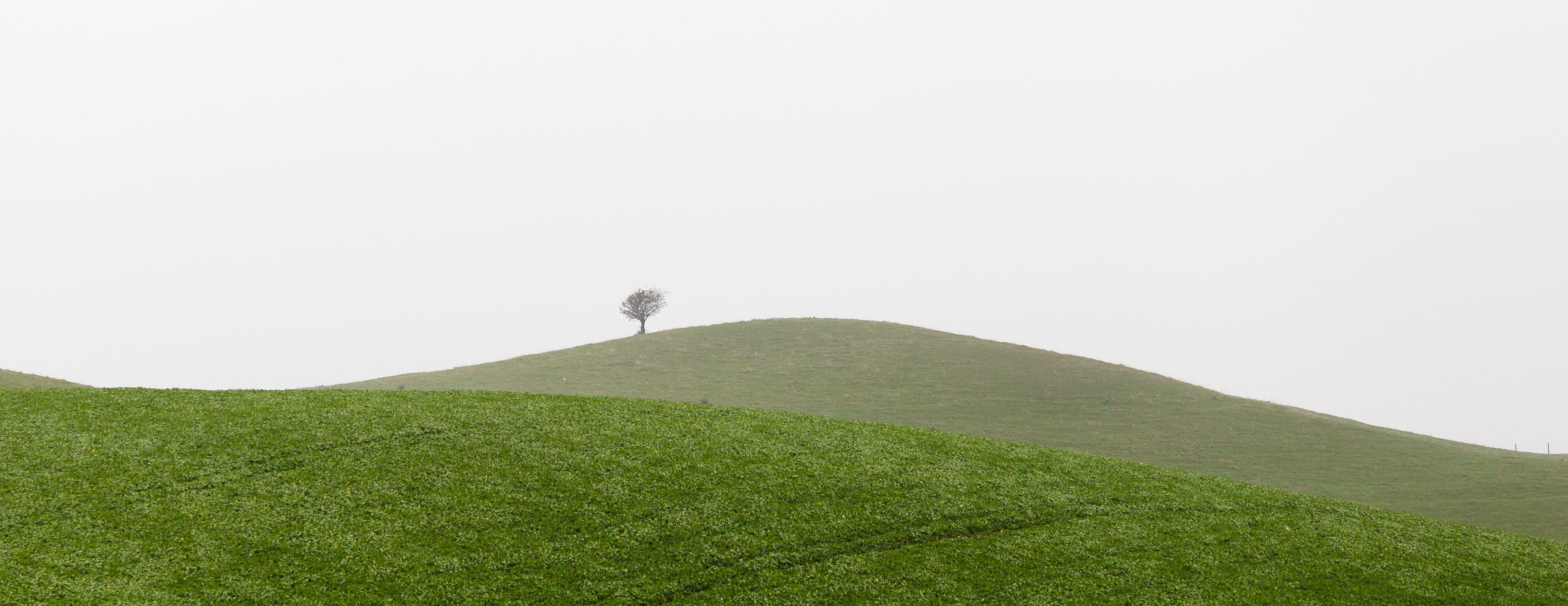
Give it a try. Press the "really wide" button on the image toolbar.
diff --git a/blocks/test/fixtures/core-image-center-caption.serialized.html b/blocks/test/fixtures/core-image-center-caption.serialized.html
index 0e3edecb381cb..b2e649e4018c4 100644
--- a/blocks/test/fixtures/core-image-center-caption.serialized.html
+++ b/blocks/test/fixtures/core-image-center-caption.serialized.html
@@ -1,5 +1,5 @@
-
+
Give it a try. Press the "really wide" button on the image toolbar.
diff --git a/blocks/test/fixtures/core-image.html b/blocks/test/fixtures/core-image.html
index 7ca7dd87f9f01..2a414855b0344 100644
--- a/blocks/test/fixtures/core-image.html
+++ b/blocks/test/fixtures/core-image.html
@@ -1,3 +1,3 @@
-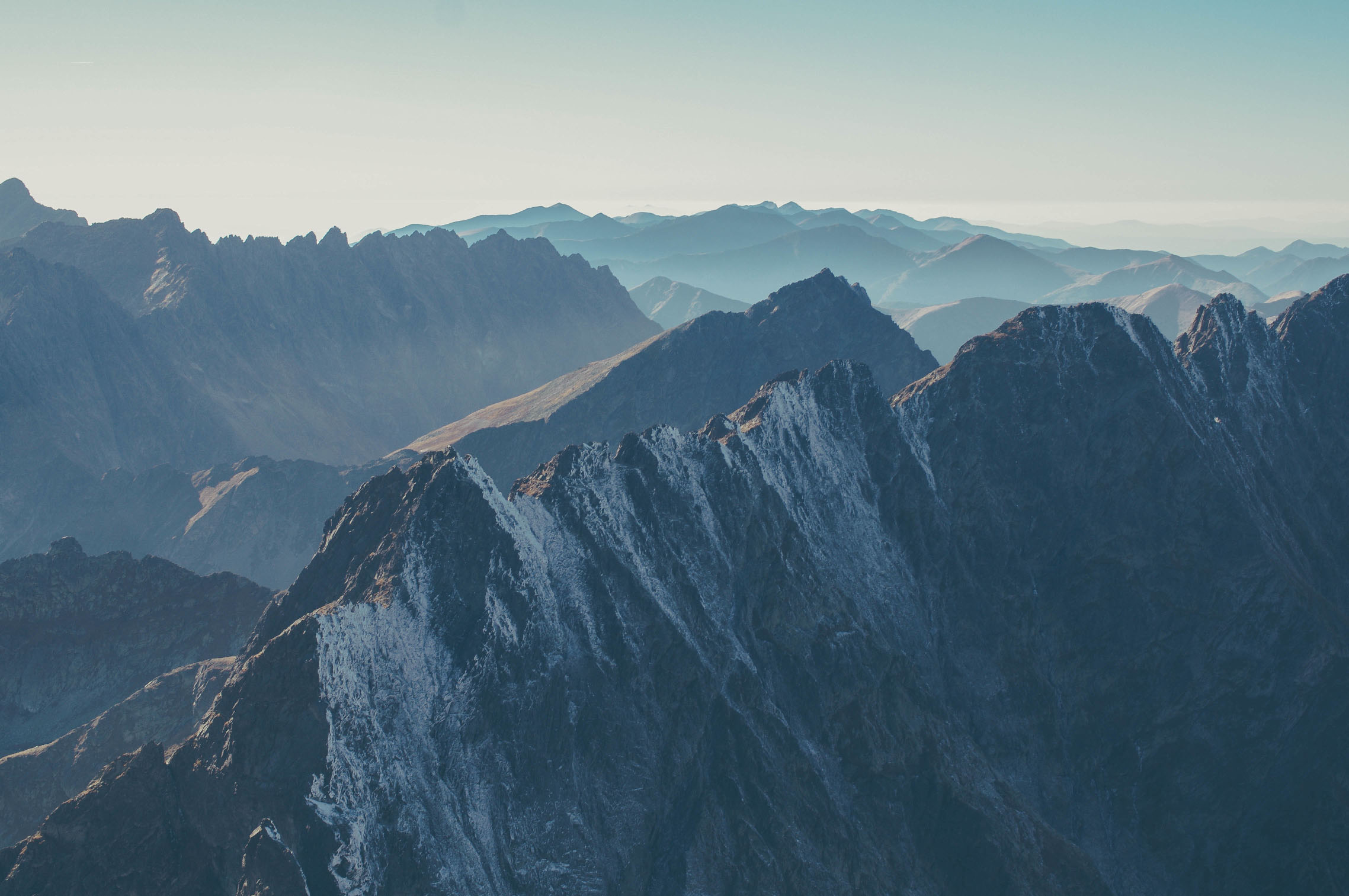
+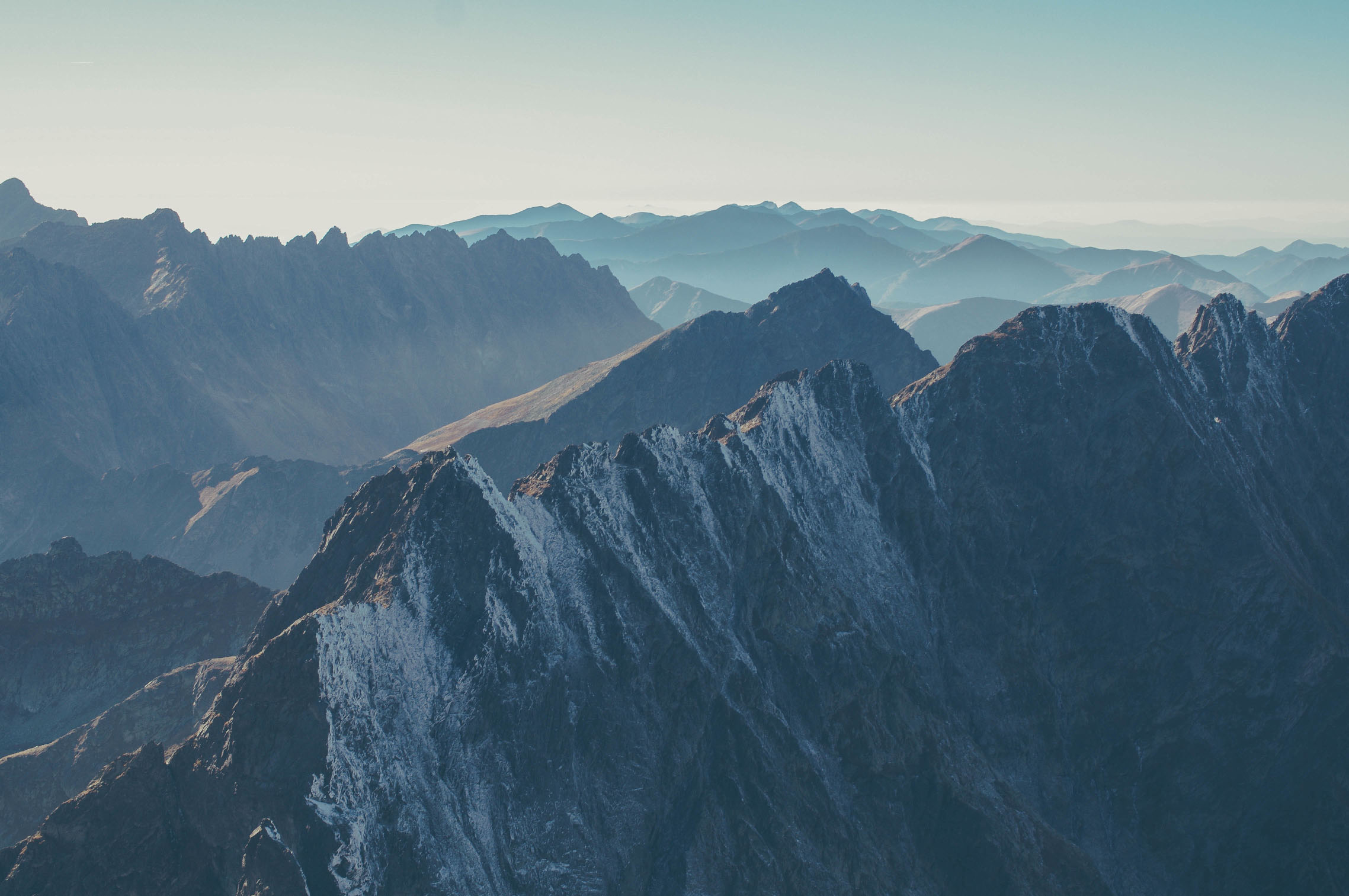
diff --git a/blocks/test/fixtures/core-image.serialized.html b/blocks/test/fixtures/core-image.serialized.html
index 2b27696131025..103b958254279 100644
--- a/blocks/test/fixtures/core-image.serialized.html
+++ b/blocks/test/fixtures/core-image.serialized.html
@@ -1,4 +1,4 @@
-
+
diff --git a/blocks/test/fixtures/core-preformatted.html b/blocks/test/fixtures/core-preformatted.html
index 1b3abc31853c0..1a890a7a5dc2d 100644
--- a/blocks/test/fixtures/core-preformatted.html
+++ b/blocks/test/fixtures/core-preformatted.html
@@ -1,3 +1,3 @@
-Some preformatted text...
And more!
+Some preformatted text...
And more!
diff --git a/blocks/test/fixtures/core-preformatted.serialized.html b/blocks/test/fixtures/core-preformatted.serialized.html
index b119b914cf0e4..ae726c700d0f1 100644
--- a/blocks/test/fixtures/core-preformatted.serialized.html
+++ b/blocks/test/fixtures/core-preformatted.serialized.html
@@ -1,4 +1,4 @@
-Some preformatted text...
And more!
+Some preformatted text...
And more!
diff --git a/blocks/test/fixtures/core-pullquote.html b/blocks/test/fixtures/core-pullquote.html
index 027188bce2960..2fa9569cddae2 100644
--- a/blocks/test/fixtures/core-pullquote.html
+++ b/blocks/test/fixtures/core-pullquote.html
@@ -1,5 +1,5 @@
-
+
Testing pullquote block...
diff --git a/blocks/test/fixtures/core-pullquote.serialized.html b/blocks/test/fixtures/core-pullquote.serialized.html
index 741f2d36a2df2..dc34d4f9689f9 100644
--- a/blocks/test/fixtures/core-pullquote.serialized.html
+++ b/blocks/test/fixtures/core-pullquote.serialized.html
@@ -1,5 +1,5 @@
-
+
Testing pullquote block...
diff --git a/blocks/test/fixtures/core-quote-style-1.html b/blocks/test/fixtures/core-quote-style-1.html
index e8c9add2748c6..a78cd61bba985 100644
--- a/blocks/test/fixtures/core-quote-style-1.html
+++ b/blocks/test/fixtures/core-quote-style-1.html
@@ -1,3 +1,3 @@
-The editor will endeavour to create a new page and post building experience that makes writing rich posts effortless, and has “blocks” to make it easy what today might take shortcodes, custom HTML, or “mystery meat” embed discovery.
+The editor will endeavour to create a new page and post building experience that makes writing rich posts effortless, and has “blocks” to make it easy what today might take shortcodes, custom HTML, or “mystery meat” embed discovery.
diff --git a/blocks/test/fixtures/core-quote-style-1.serialized.html b/blocks/test/fixtures/core-quote-style-1.serialized.html
index e38001b88f29a..b89cf527cb38c 100644
--- a/blocks/test/fixtures/core-quote-style-1.serialized.html
+++ b/blocks/test/fixtures/core-quote-style-1.serialized.html
@@ -1,5 +1,5 @@
-
+
The editor will endeavour to create a new page and post building experience that makes writing rich posts effortless, and has “blocks” to make it easy what today might take shortcodes, custom HTML, or “mystery meat” embed discovery.
diff --git a/blocks/test/fixtures/core-quote-style-2.html b/blocks/test/fixtures/core-quote-style-2.html
index b8c255beee8fe..5770cb815e678 100644
--- a/blocks/test/fixtures/core-quote-style-2.html
+++ b/blocks/test/fixtures/core-quote-style-2.html
@@ -1,3 +1,3 @@
-There is no greater agony than bearing an untold story inside you.
+There is no greater agony than bearing an untold story inside you.
diff --git a/blocks/test/fixtures/core-quote-style-2.serialized.html b/blocks/test/fixtures/core-quote-style-2.serialized.html
index 6d5b9988eb347..94750496b9a4f 100644
--- a/blocks/test/fixtures/core-quote-style-2.serialized.html
+++ b/blocks/test/fixtures/core-quote-style-2.serialized.html
@@ -1,5 +1,5 @@
-
+
There is no greater agony than bearing an untold story inside you.
diff --git a/blocks/test/fixtures/core-separator.html b/blocks/test/fixtures/core-separator.html
index a241e07204f9a..d835a483147f1 100644
--- a/blocks/test/fixtures/core-separator.html
+++ b/blocks/test/fixtures/core-separator.html
@@ -1,3 +1,3 @@
-
+
diff --git a/blocks/test/fixtures/core-separator.serialized.html b/blocks/test/fixtures/core-separator.serialized.html
index a411529200442..0cc337d26dc88 100644
--- a/blocks/test/fixtures/core-separator.serialized.html
+++ b/blocks/test/fixtures/core-separator.serialized.html
@@ -1,4 +1,4 @@
-
+
diff --git a/blocks/test/fixtures/core-table.html b/blocks/test/fixtures/core-table.html
index 60008a1566ef0..de0498f605be4 100644
--- a/blocks/test/fixtures/core-table.html
+++ b/blocks/test/fixtures/core-table.html
@@ -1,5 +1,5 @@
-
+
Version
diff --git a/blocks/test/fixtures/core-table.serialized.html b/blocks/test/fixtures/core-table.serialized.html
index 174adfec5ae84..40d9109b06271 100644
--- a/blocks/test/fixtures/core-table.serialized.html
+++ b/blocks/test/fixtures/core-table.serialized.html
@@ -1,5 +1,5 @@
-
+
Version
diff --git a/post-content.js b/post-content.js
index 3f4b930161869..17d8d6b298329 100644
--- a/post-content.js
+++ b/post-content.js
@@ -9,7 +9,7 @@ window._wpGutenbergPost = {
content: {
raw: [
'',
- 'Gutenberg Editor
',
+ 'Gutenberg Editor
',
'',
'',
@@ -39,7 +39,7 @@ window._wpGutenbergPost = {
'',
'',
- '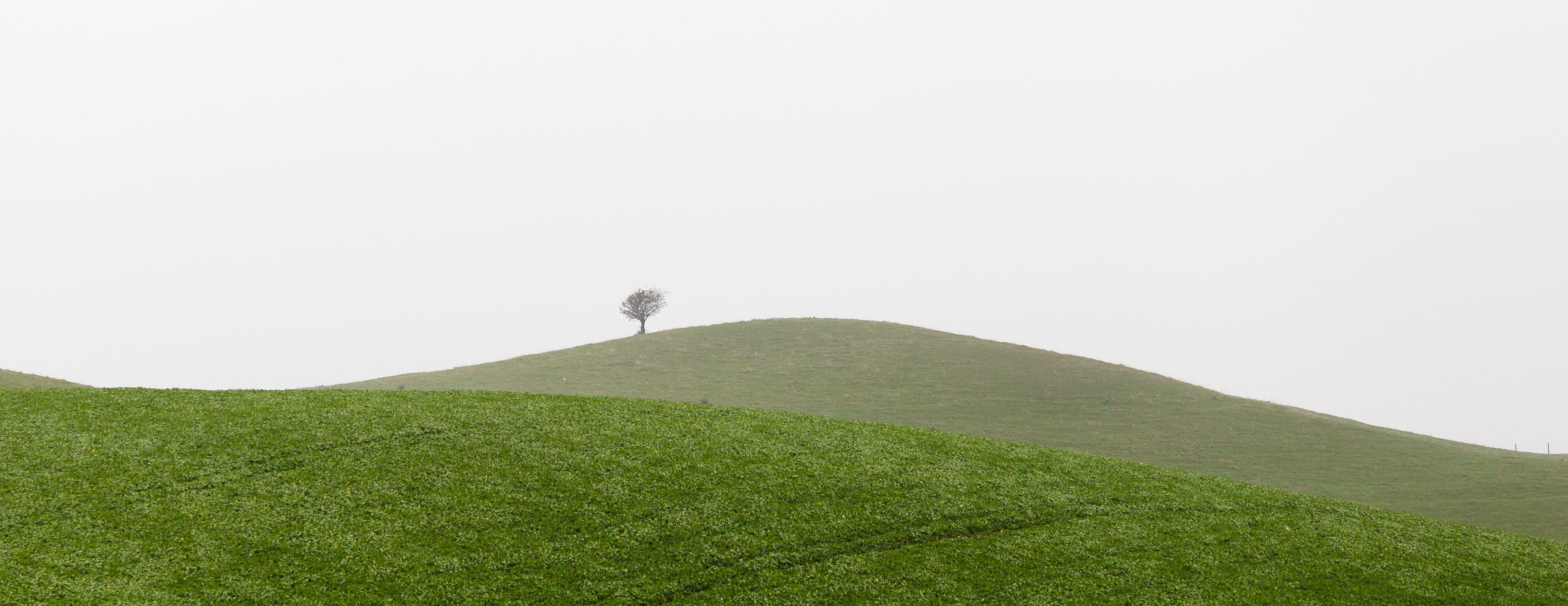
Give it a try. Press the "really wide" button on the image toolbar. ',
+ '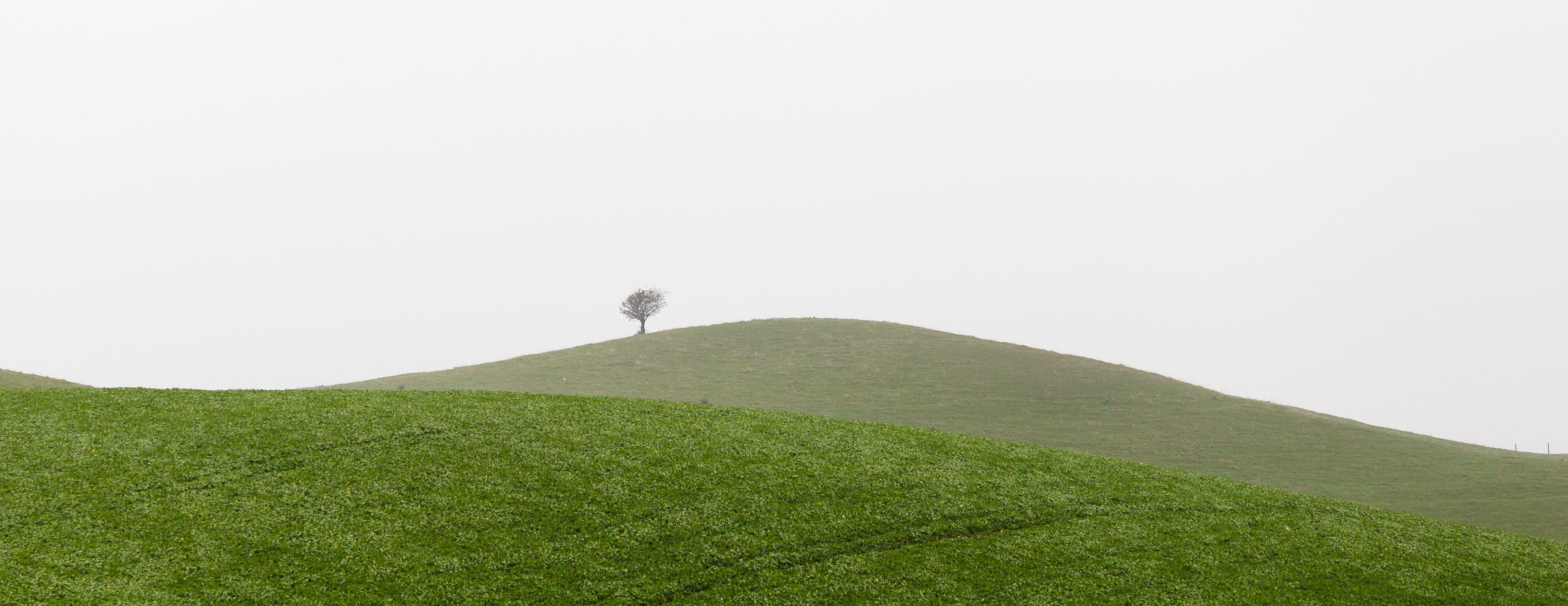
Give it a try. Press the "really wide" button on the image toolbar. ',
'',
'',
@@ -67,11 +67,11 @@ window._wpGutenbergPost = {
'',
'',
- '',
+ ' ',
'',
'',
- '
',
+ '
',
'',
'',
@@ -83,7 +83,7 @@ window._wpGutenbergPost = {
'',
'',
- 'The editor will endeavour to create a new page and post building experience that makes writing rich posts effortless, and has “blocks” to make it easy what today might take shortcodes, custom HTML, or “mystery meat” embed discovery.
',
+ 'The editor will endeavour to create a new page and post building experience that makes writing rich posts effortless, and has “blocks” to make it easy what today might take shortcodes, custom HTML, or “mystery meat” embed discovery.
',
'',
'',
@@ -95,11 +95,11 @@ window._wpGutenbergPost = {
'',
'',
- 'There is no greater agony than bearing an untold story inside you.
',
+ 'There is no greater agony than bearing an untold story inside you.
',
'',
'',
- '
',
+ '
',
'',
'',
@@ -111,7 +111,7 @@ window._wpGutenbergPost = {
'',
'',
- '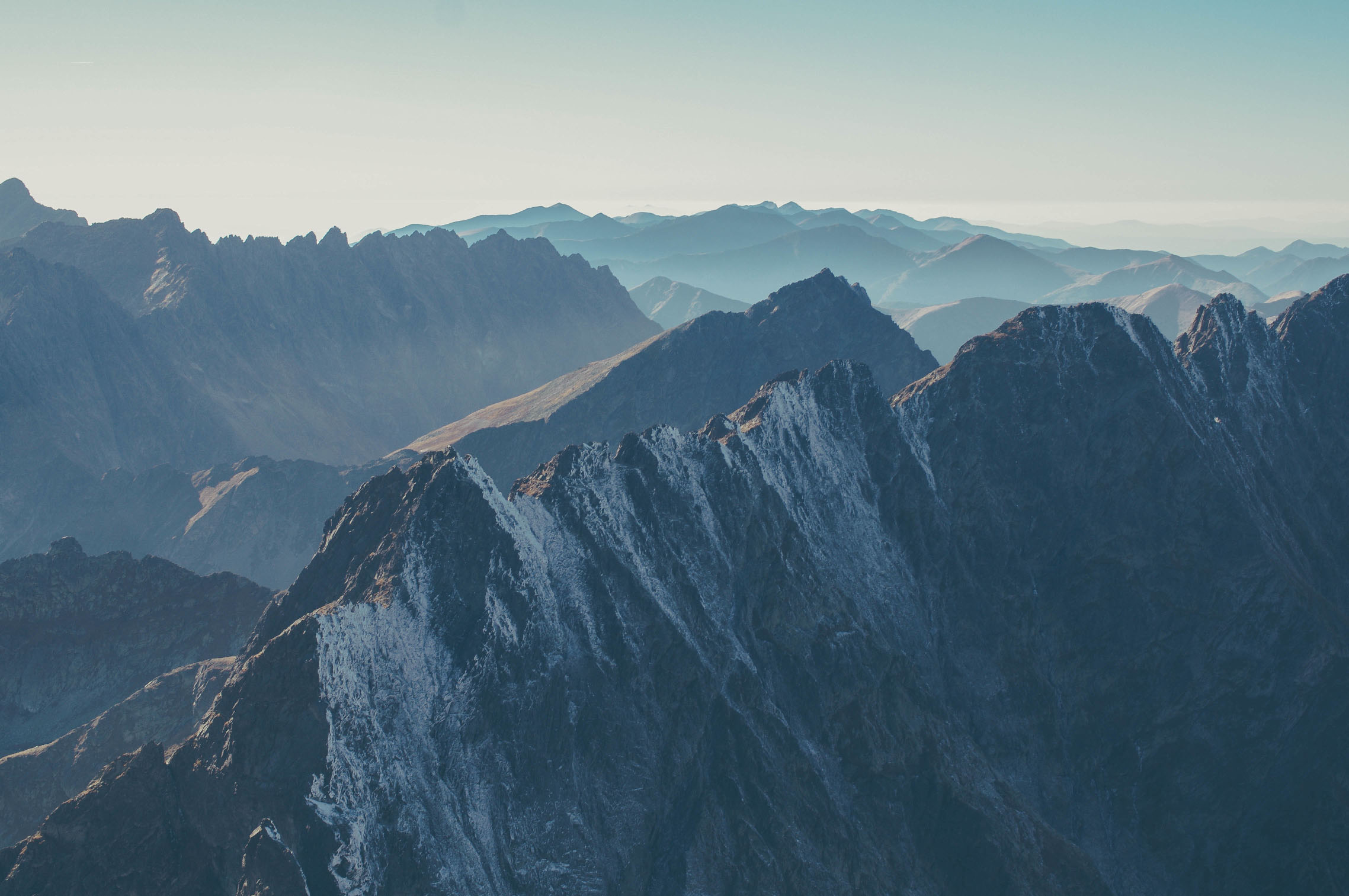
',
+ '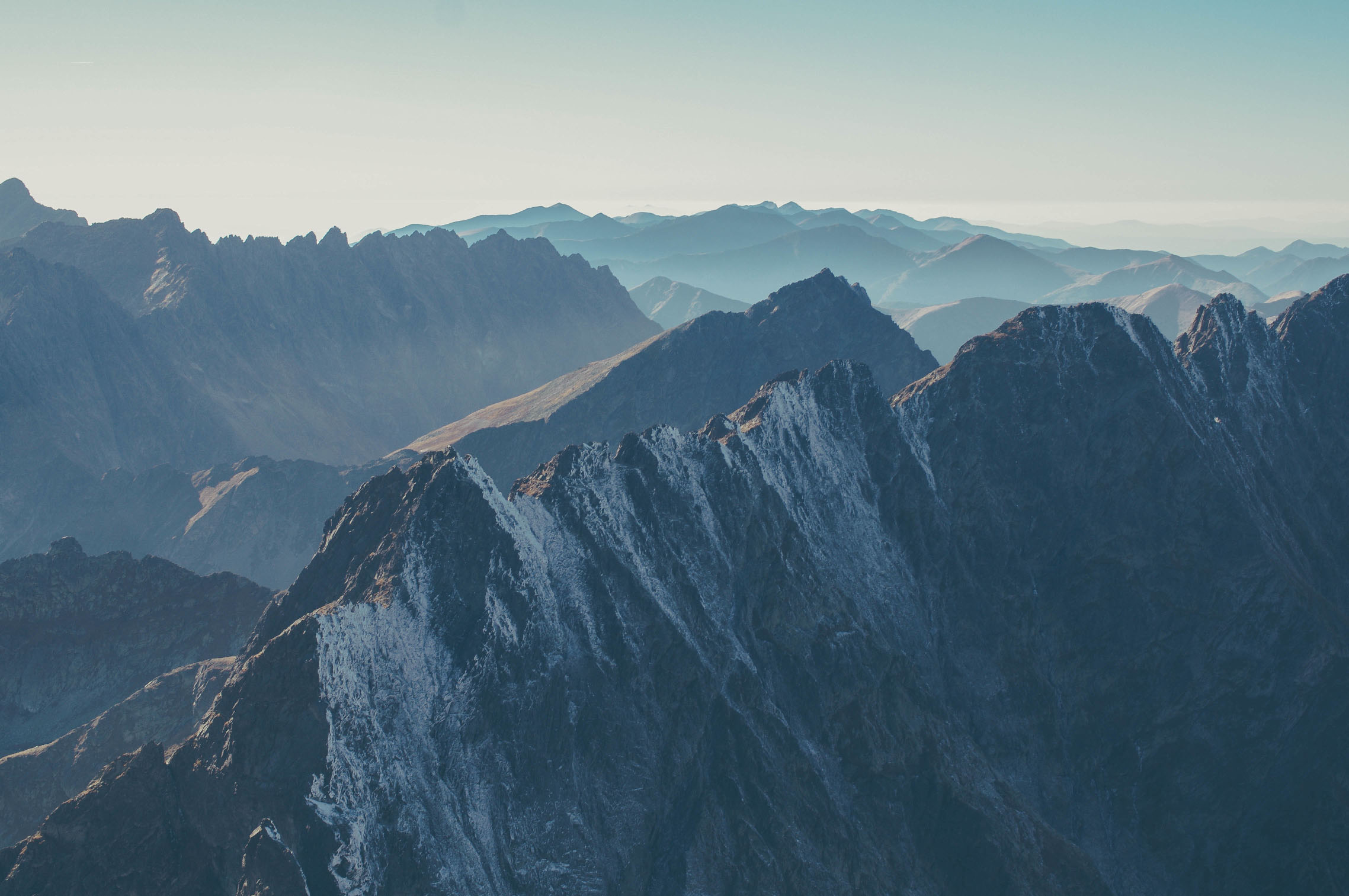
',
'',
'',
@@ -123,21 +123,21 @@ window._wpGutenbergPost = {
'',
'',
- 'export default function MyButton() {\n\
+ 'export default function MyButton() {\n\
return <Button>Click Me!</Button>;\n\
}
',
'',
'',
- '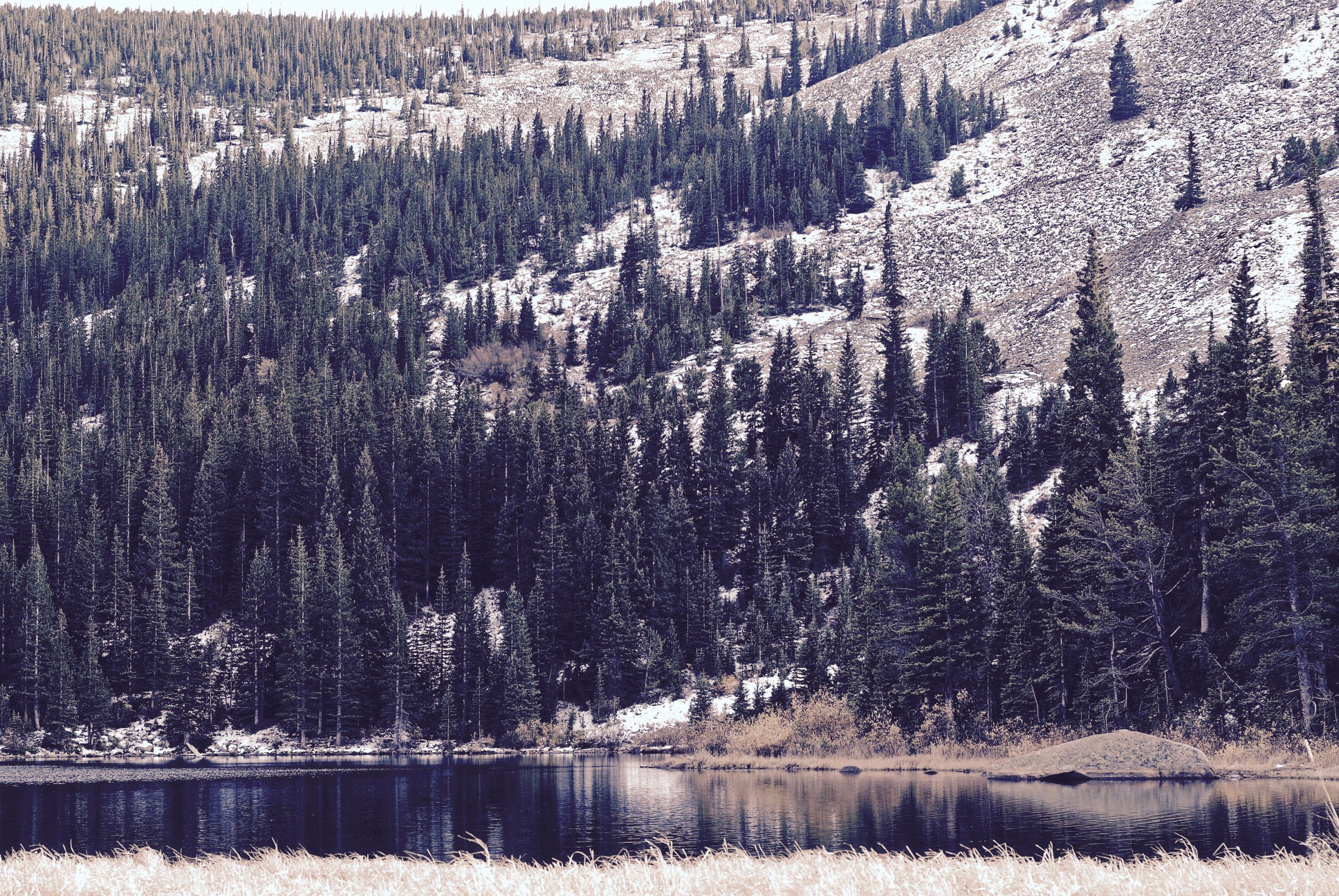
',
+ '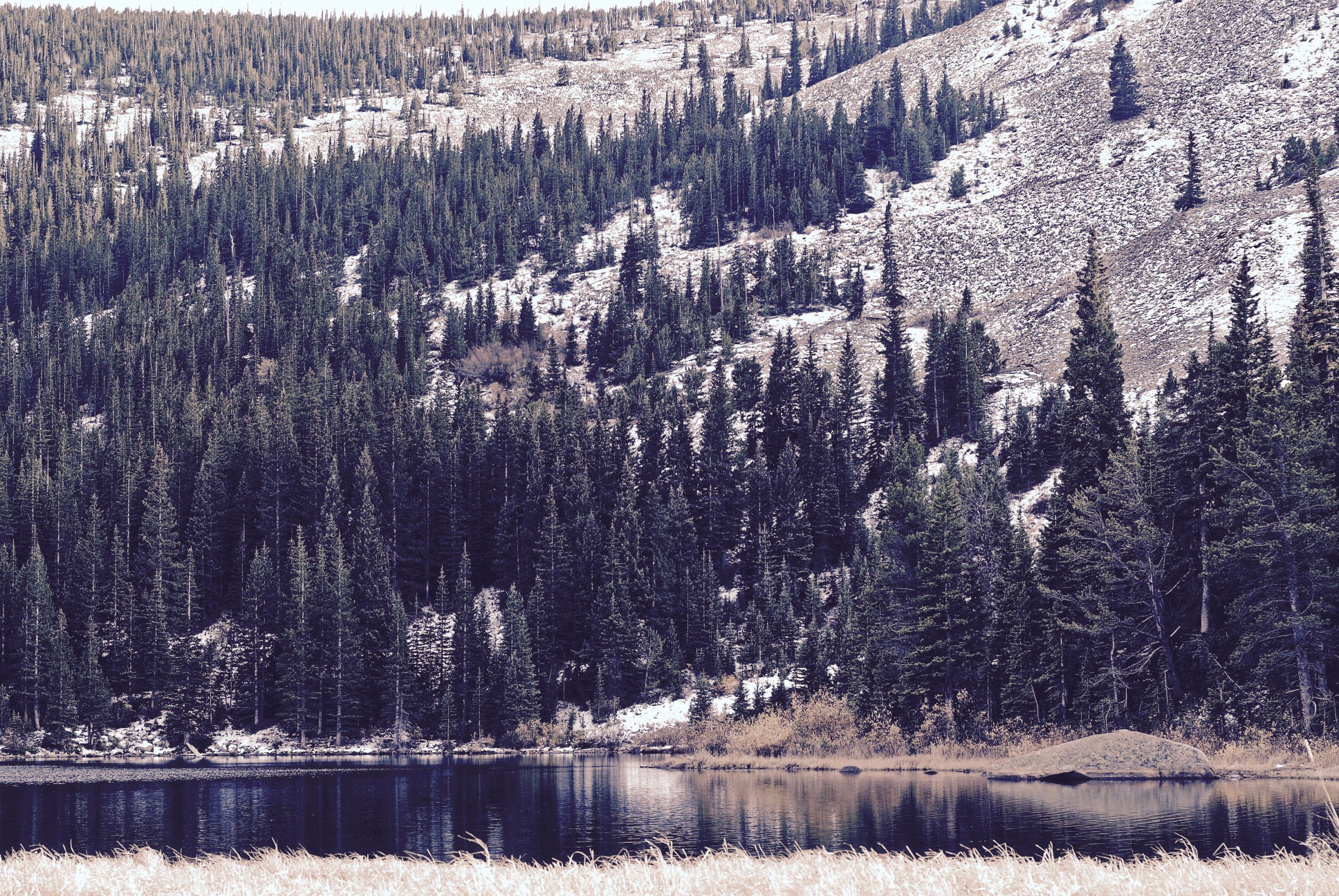
',
'',
'',
- '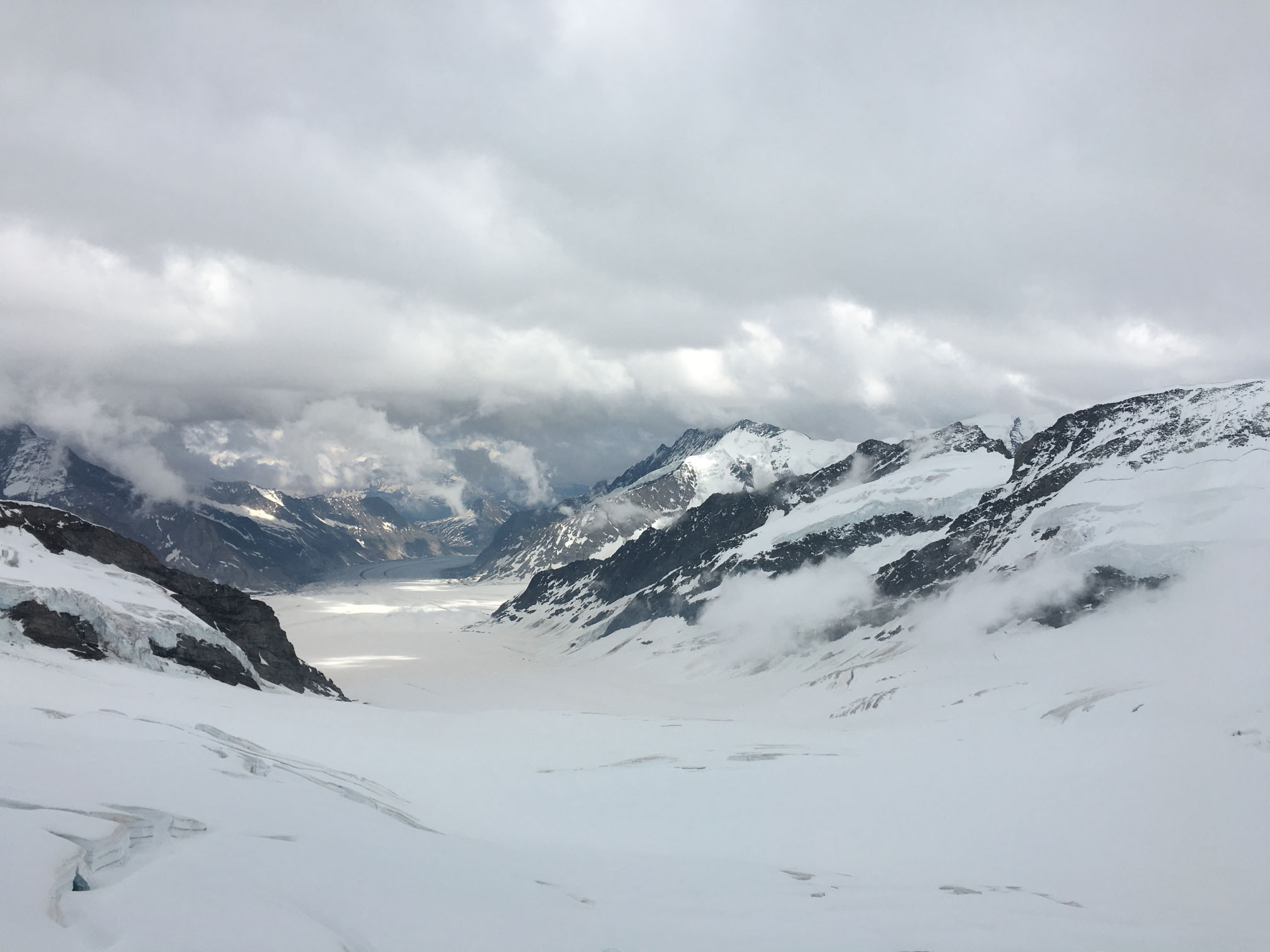
',
+ '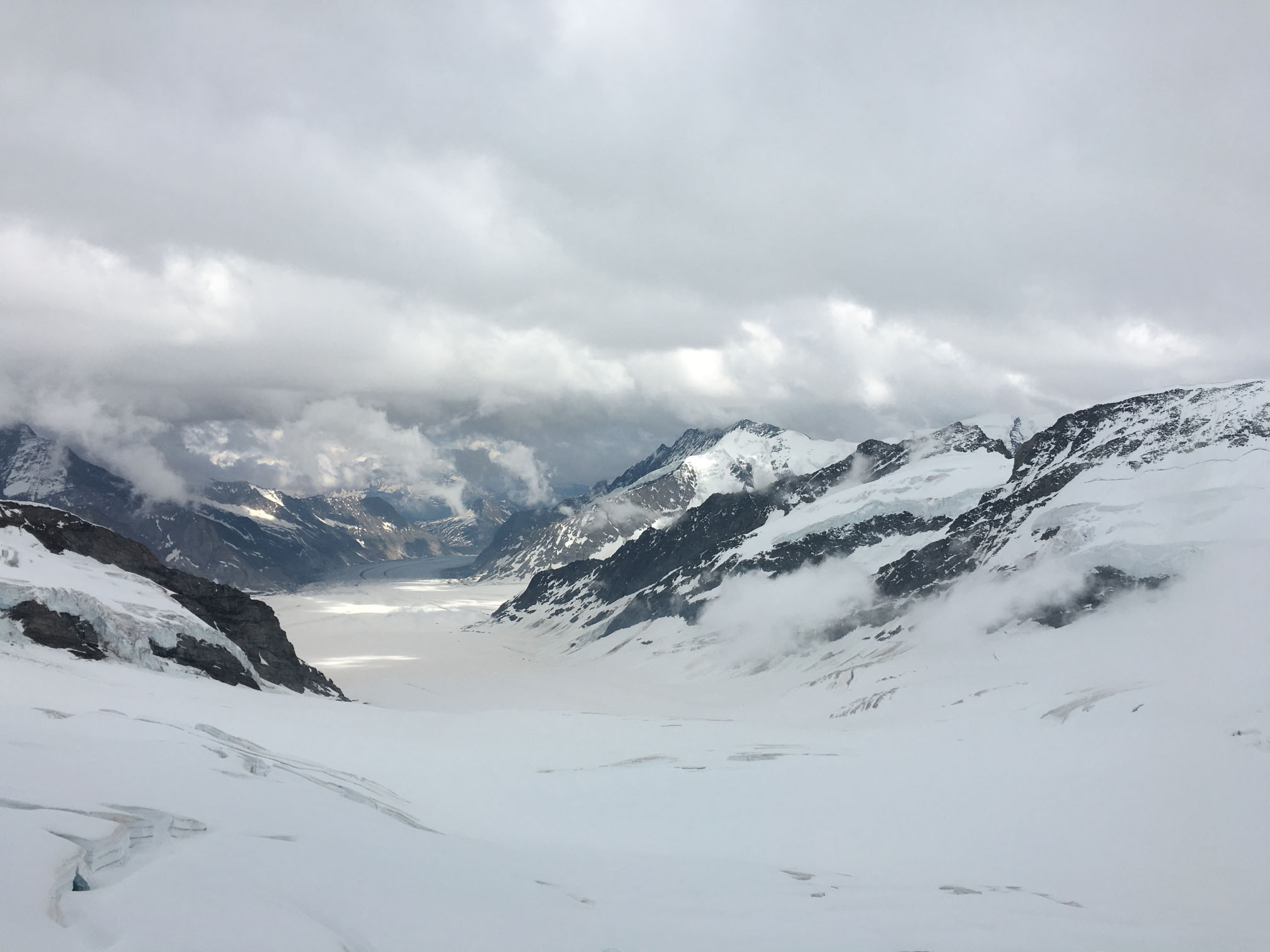
',
'',
'',
- 'An old silent pond...
A frog jumps into the pond,
splash! Silence again.
',
+ 'An old silent pond...
A frog jumps into the pond,
splash! Silence again.
',
'',
'',
@@ -153,15 +153,15 @@ window._wpGutenbergPost = {
'',
'',
- 'Code is Poetry
',
+ 'Code is Poetry
',
'',
'',
- '
',
+ '
',
'',
'',
- 'Version Musician Date 4.4 Clifford Brown December 8, 2015 4.5 Coleman Hawkins April 12, 2016 4.6 Pepper Adams August 16, 2016 4.7 Sarah Vaughan December 6, 2016
',
+ 'Version Musician Date 4.4 Clifford Brown December 8, 2015 4.5 Coleman Hawkins April 12, 2016 4.6 Pepper Adams August 16, 2016 4.7 Sarah Vaughan December 6, 2016
',
'',
'',
@@ -169,7 +169,7 @@ window._wpGutenbergPost = {
'',
'',
- 'https://www.youtube.com/watch?v=Nl6U7UotA-MState of the Word 2016 ',
+ 'https://www.youtube.com/watch?v=Nl6U7UotA-MState of the Word 2016 ',
'',
'',