+
diff --git a/blocks/library/gallery/index.js b/blocks/library/gallery/index.js
index 5d208791144231..a891e21969de1e 100644
--- a/blocks/library/gallery/index.js
+++ b/blocks/library/gallery/index.js
@@ -17,51 +17,52 @@ import './style.scss';
import { registerBlockType, createBlock } from '../../api';
import { default as GalleryBlock, defaultColumnsNumber } from './block';
+const blockAttributes = {
+ align: {
+ type: 'string',
+ default: 'none',
+ },
+ images: {
+ type: 'array',
+ default: [],
+ source: 'query',
+ selector: 'ul.wp-block-gallery .blocks-gallery-item img',
+ query: {
+ url: {
+ source: 'attribute',
+ attribute: 'src',
+ },
+ alt: {
+ source: 'attribute',
+ attribute: 'alt',
+ default: '',
+ },
+ id: {
+ source: 'attribute',
+ attribute: 'data-id',
+ },
+ },
+ },
+ columns: {
+ type: 'number',
+ },
+ imageCrop: {
+ type: 'boolean',
+ default: true,
+ },
+ linkTo: {
+ type: 'string',
+ default: 'none',
+ },
+};
+
registerBlockType( 'core/gallery', {
title: __( 'Gallery' ),
description: __( 'Image galleries are a great way to share groups of pictures on your site.' ),
icon: 'format-gallery',
category: 'common',
keywords: [ __( 'images' ), __( 'photos' ) ],
-
- attributes: {
- align: {
- type: 'string',
- default: 'none',
- },
- images: {
- type: 'array',
- default: [],
- source: 'query',
- selector: 'div.wp-block-gallery figure.blocks-gallery-image img',
- query: {
- url: {
- source: 'attribute',
- attribute: 'src',
- },
- alt: {
- source: 'attribute',
- attribute: 'alt',
- default: '',
- },
- id: {
- source: 'attribute',
- attribute: 'data-id',
- },
- },
- },
- columns: {
- type: 'number',
- },
- imageCrop: {
- type: 'boolean',
- default: true,
- },
- linkTo: {
- type: 'string',
- default: 'none',
- },
- },
+ attributes: blockAttributes,
transforms: {
from: [
@@ -69,8 +70,8 @@ registerBlockType( 'core/gallery', {
type: 'block',
isMultiBlock: true,
blocks: [ 'core/image' ],
- transform: ( blockAttributes ) => {
- const validImages = filter( blockAttributes, ( { id, url } ) => id && url );
+ transform: ( attributes ) => {
+ const validImages = filter( attributes, ( { id, url } ) => id && url );
if ( validImages.length > 0 ) {
return createBlock( 'core/gallery', {
images: validImages.map( ( { id, url, alt } ) => ( { id, url, alt } ) ),
@@ -151,7 +152,7 @@ registerBlockType( 'core/gallery', {
save( { attributes } ) {
const { images, columns = defaultColumnsNumber( attributes ), align, imageCrop, linkTo } = attributes;
return (
-
+
{ images.map( ( image ) => {
let href;
@@ -167,13 +168,55 @@ registerBlockType( 'core/gallery', {
const img =
;
return (
-
- { href ? { img } : img }
-
+ -
+
+ { href ? { img } : img }
+
+
);
} ) }
-
+
);
},
+ deprecated: [
+ {
+ attributes: {
+ ...blockAttributes,
+ images: {
+ ...blockAttributes.images,
+ selector: 'div.wp-block-gallery figure.blocks-gallery-image img',
+ },
+ },
+
+ save( { attributes } ) {
+ const { images, columns = defaultColumnsNumber( attributes ), align, imageCrop, linkTo } = attributes;
+ return (
+
+ { images.map( ( image ) => {
+ let href;
+
+ switch ( linkTo ) {
+ case 'media':
+ href = image.url;
+ break;
+ case 'attachment':
+ href = image.link;
+ break;
+ }
+
+ const img =

;
+
+ return (
+
+ { href ? { img } : img }
+
+ );
+ } ) }
+
+ );
+ },
+ },
+ ],
+
} );
diff --git a/blocks/library/gallery/style.scss b/blocks/library/gallery/style.scss
index 7f4e436eaa2d5f..96b4f3d5081196 100644
--- a/blocks/library/gallery/style.scss
+++ b/blocks/library/gallery/style.scss
@@ -4,22 +4,31 @@
.wp-block-gallery.aligncenter {
display: flex;
flex-wrap: wrap;
+ list-style-type: none;
- .blocks-gallery-image {
+ .blocks-gallery-image,
+ .blocks-gallery-item {
margin: 8px;
display: flex;
flex-grow: 1;
flex-direction: column;
justify-content: center;
+ figure {
+ height: 100%;
+ margin: 0;
+ }
+
img {
+ display: block;
max-width: 100%;
height: auto;
}
}
// Cropped
- &.is-cropped .blocks-gallery-image {
+ &.is-cropped .blocks-gallery-image,
+ &.is-cropped .blocks-gallery-item {
img {
flex: 1;
width: 100%;
@@ -29,47 +38,29 @@
}
// Alas, IE11+ doesn't support object-fit
- _:-ms-lang(x), img {
+ _:-ms-lang(x), figure {
height: auto;
width: auto;
}
}
- &.columns-1 figure {
- width: calc(100% / 1 - 16px);
- }
- &.columns-2 figure {
- width: calc(100% / 2 - 16px);
+ // Responsive fallback value, 2 columns
+ & .blocks-gallery-image,
+ & .blocks-gallery-item {
+ width: calc( 100% / 2 - 16px );
}
- // Responsive fallback value, 2 columns
- &.columns-3 figure,
- &.columns-4 figure,
- &.columns-5 figure,
- &.columns-6 figure,
- &.columns-7 figure,
- &.columns-8 figure {
- width: calc(100% / 2 - 16px);
+ &.columns-1 .blocks-gallery-image,
+ &.columns-1 .blocks-gallery-item {
+ width: calc(100% / 1 - 16px);
}
@include break-small {
- &.columns-3 figure {
- width: calc(100% / 3 - 16px);
- }
- &.columns-4 figure {
- width: calc(100% / 4 - 16px);
- }
- &.columns-5 figure {
- width: calc(100% / 5 - 16px);
- }
- &.columns-6 figure {
- width: calc(100% / 6 - 16px);
- }
- &.columns-7 figure {
- width: calc(100% / 7 - 16px);
- }
- &.columns-8 figure {
- width: calc(100% / 8 - 16px);
+ @for $i from 3 through 8 {
+ &.columns-#{ $i } .blocks-gallery-image,
+ &.columns-#{ $i } .blocks-gallery-item {
+ width: calc(100% / #{ $i } - 16px );
+ }
}
}
}
diff --git a/blocks/test/fixtures/core__gallery.html b/blocks/test/fixtures/core__gallery.html
index b74b304f41e3f3..ec923b2a360432 100644
--- a/blocks/test/fixtures/core__gallery.html
+++ b/blocks/test/fixtures/core__gallery.html
@@ -1,10 +1,14 @@
-
-
-
-
-
-
-
-
+
+ -
+
+
+
+
+ -
+
+
+
+
+
diff --git a/blocks/test/fixtures/core__gallery.json b/blocks/test/fixtures/core__gallery.json
index 4d1777693f7532..60f85dd327fe71 100644
--- a/blocks/test/fixtures/core__gallery.json
+++ b/blocks/test/fixtures/core__gallery.json
@@ -18,6 +18,6 @@
"imageCrop": true,
"linkTo": "none"
},
- "originalContent": "
\n\t
\n\t\t
\n\t\n\t
\n\t\t
\n\t\n
"
+ "originalContent": "
\n\t- \n\t\t\n\t\t\t
\n\t\t\n\t \n\t- \n\t\t\n\t\t\t
\n\t\t\n\t \n
"
}
]
diff --git a/blocks/test/fixtures/core__gallery.parsed.json b/blocks/test/fixtures/core__gallery.parsed.json
index f4c318b6e5213b..e187acdd51e60d 100644
--- a/blocks/test/fixtures/core__gallery.parsed.json
+++ b/blocks/test/fixtures/core__gallery.parsed.json
@@ -3,7 +3,7 @@
"blockName": "core/gallery",
"attrs": null,
"innerBlocks": [],
- "innerHTML": "\n
\n\t
\n\t\t
\n\t\n\t
\n\t\t
\n\t\n
\n"
+ "innerHTML": "\n
\n\t- \n\t\t\n\t\t\t
\n\t\t\n\t \n\t- \n\t\t\n\t\t\t
\n\t\t\n\t \n
\n"
},
{
"attrs": {},
diff --git a/blocks/test/fixtures/core__gallery.serialized.html b/blocks/test/fixtures/core__gallery.serialized.html
index f00d97942ebef8..2e93e66f6c4ae7 100644
--- a/blocks/test/fixtures/core__gallery.serialized.html
+++ b/blocks/test/fixtures/core__gallery.serialized.html
@@ -1,6 +1,10 @@
-
-
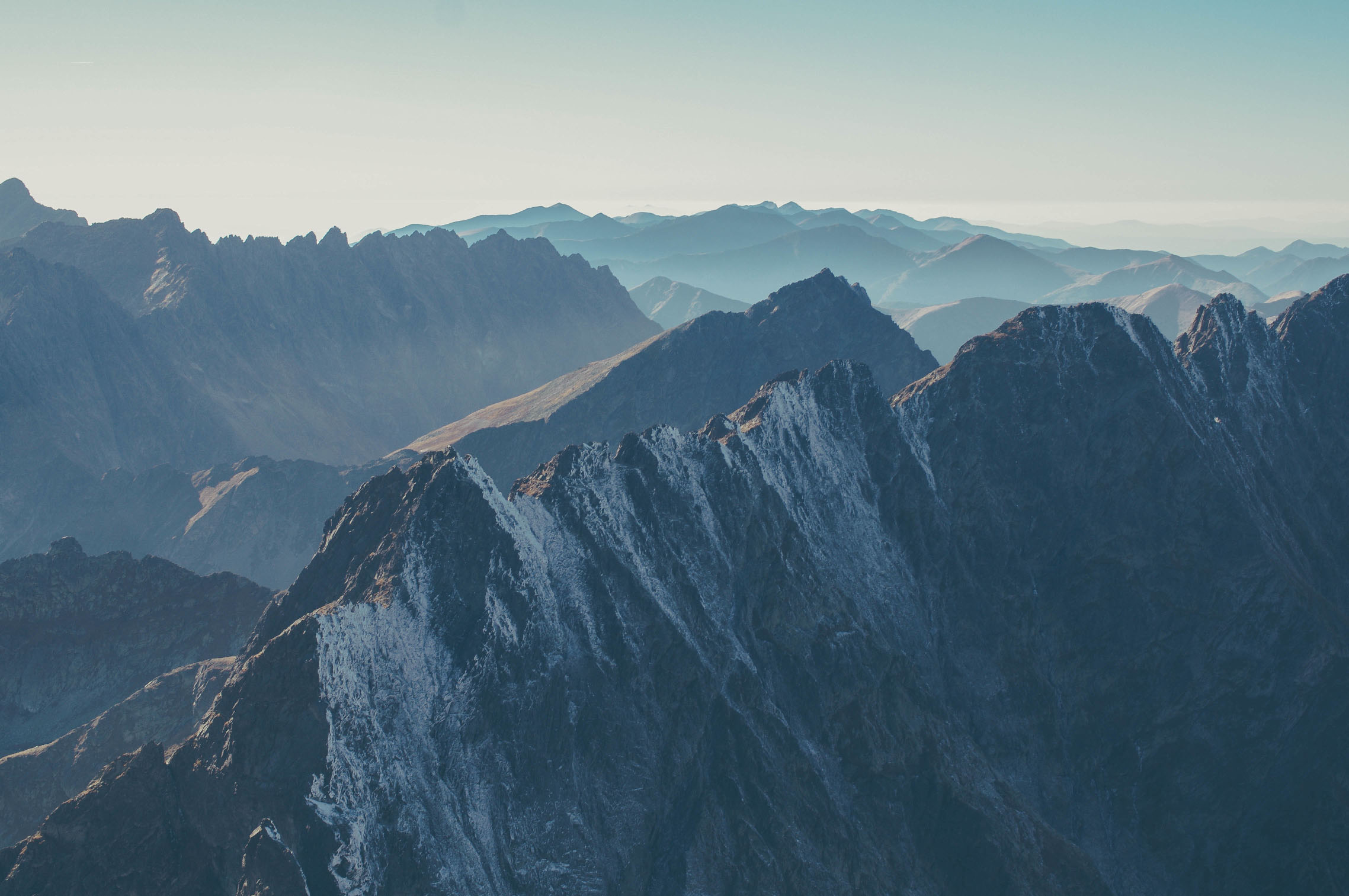
-

-
+
+ -
+
+
+ -
+
+
+
diff --git a/blocks/test/fixtures/core__gallery__columns.html b/blocks/test/fixtures/core__gallery__columns.html
index 3fe69778320f66..24d87615b81a69 100644
--- a/blocks/test/fixtures/core__gallery__columns.html
+++ b/blocks/test/fixtures/core__gallery__columns.html
@@ -1,10 +1,14 @@
-
-
-
-
-
-
-
-
+
+ -
+
+
+
+
+ -
+
+
+
+
+
diff --git a/blocks/test/fixtures/core__gallery__columns.json b/blocks/test/fixtures/core__gallery__columns.json
index 2b3e2e7fca6e6f..d5444c63be8a19 100644
--- a/blocks/test/fixtures/core__gallery__columns.json
+++ b/blocks/test/fixtures/core__gallery__columns.json
@@ -19,6 +19,6 @@
"imageCrop": true,
"linkTo": "none"
},
- "originalContent": "
\n\t
\n\t\t
\n\t\n\t
\n\t\t
\n\t\n
"
+ "originalContent": "
\n\t- \n\t\t\n\t\t\t
\n\t\t\n\t \n\t- \n\t\t\n\t\t\t
\n\t\t\n\t \n
"
}
]
diff --git a/blocks/test/fixtures/core__gallery__columns.parsed.json b/blocks/test/fixtures/core__gallery__columns.parsed.json
index 291420ff0b4c3e..8fd100edece694 100644
--- a/blocks/test/fixtures/core__gallery__columns.parsed.json
+++ b/blocks/test/fixtures/core__gallery__columns.parsed.json
@@ -5,7 +5,7 @@
"columns": "1"
},
"innerBlocks": [],
- "innerHTML": "\n
\n\t
\n\t\t
\n\t\n\t
\n\t\t
\n\t\n
\n"
+ "innerHTML": "\n
\n\t- \n\t\t\n\t\t\t
\n\t\t\n\t \n\t- \n\t\t\n\t\t\t
\n\t\t\n\t \n
\n"
},
{
"attrs": {},
diff --git a/blocks/test/fixtures/core__gallery__columns.serialized.html b/blocks/test/fixtures/core__gallery__columns.serialized.html
index 39501c3ae1a1e3..63a881085e213f 100644
--- a/blocks/test/fixtures/core__gallery__columns.serialized.html
+++ b/blocks/test/fixtures/core__gallery__columns.serialized.html
@@ -1,6 +1,10 @@
-
-
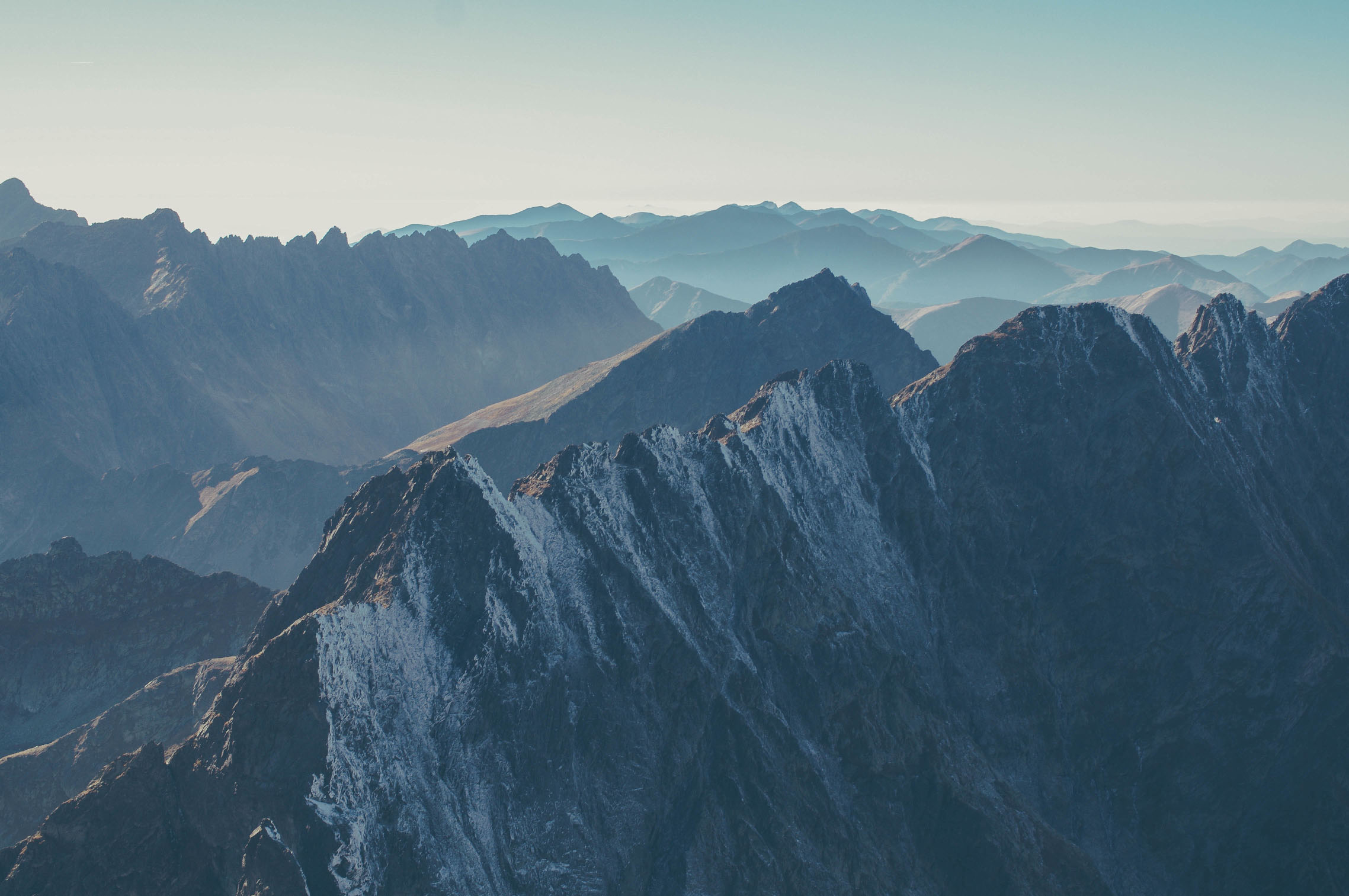
-

-
+
+ -
+
+
+ -
+
+
+
diff --git a/editor/assets/stylesheets/_z-index.scss b/editor/assets/stylesheets/_z-index.scss
index f218da7a81da5f..6ddcf087abb80a 100644
--- a/editor/assets/stylesheets/_z-index.scss
+++ b/editor/assets/stylesheets/_z-index.scss
@@ -22,7 +22,7 @@ $z-layers: (
'.editor-block-switcher__menu': 2,
'.components-popover__close': 2,
'.editor-block-mover': 1,
- '.blocks-gallery-image__inline-menu': 20,
+ '.blocks-gallery-item__inline-menu': 20,
'.editor-block-settings-menu__popover': 20, // Below the header
'.editor-header': 30,
'.editor-text-editor__formatting': 30,
diff --git a/editor/assets/stylesheets/main.scss b/editor/assets/stylesheets/main.scss
index b01d63b1bb69b8..6c9256ad6f0456 100644
--- a/editor/assets/stylesheets/main.scss
+++ b/editor/assets/stylesheets/main.scss
@@ -43,11 +43,11 @@ body.gutenberg-editor-page {
box-sizing: border-box;
}
- ul {
+ ul:not(.wp-block-gallery) {
list-style-type: disc;
}
- ol {
+ ol:not(.wp-block-gallery) {
list-style-type: decimal;
}
diff --git a/phpunit/fixtures/long-content.html b/phpunit/fixtures/long-content.html
index 35194ee4770307..d4b935b510513f 100644
--- a/phpunit/fixtures/long-content.html
+++ b/phpunit/fixtures/long-content.html
@@ -67,9 +67,9 @@
Visual Editing
@@ -89,8 +89,8 @@
Media Rich
diff --git a/post-content.js b/post-content.js
index aec808790da651..7c3cd133ea6f4c 100644
--- a/post-content.js
+++ b/post-content.js
@@ -84,11 +84,11 @@ window._wpGutenbergPost.content = {
'',
'',
- '
',
+ '
',
'',
'',
@@ -112,10 +112,10 @@ window._wpGutenbergPost.content = {
'',
'',
- '
',
- '
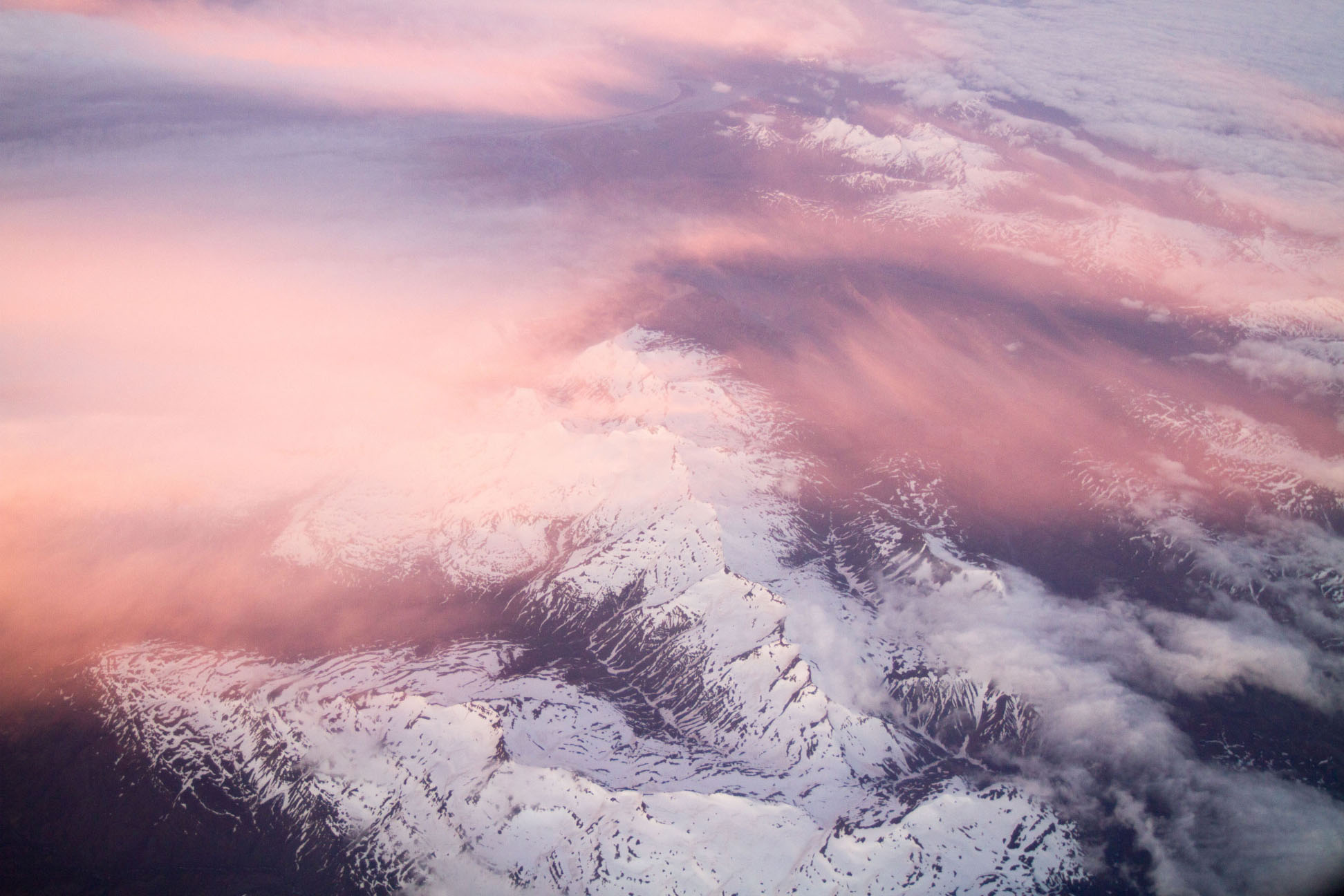
',
- '
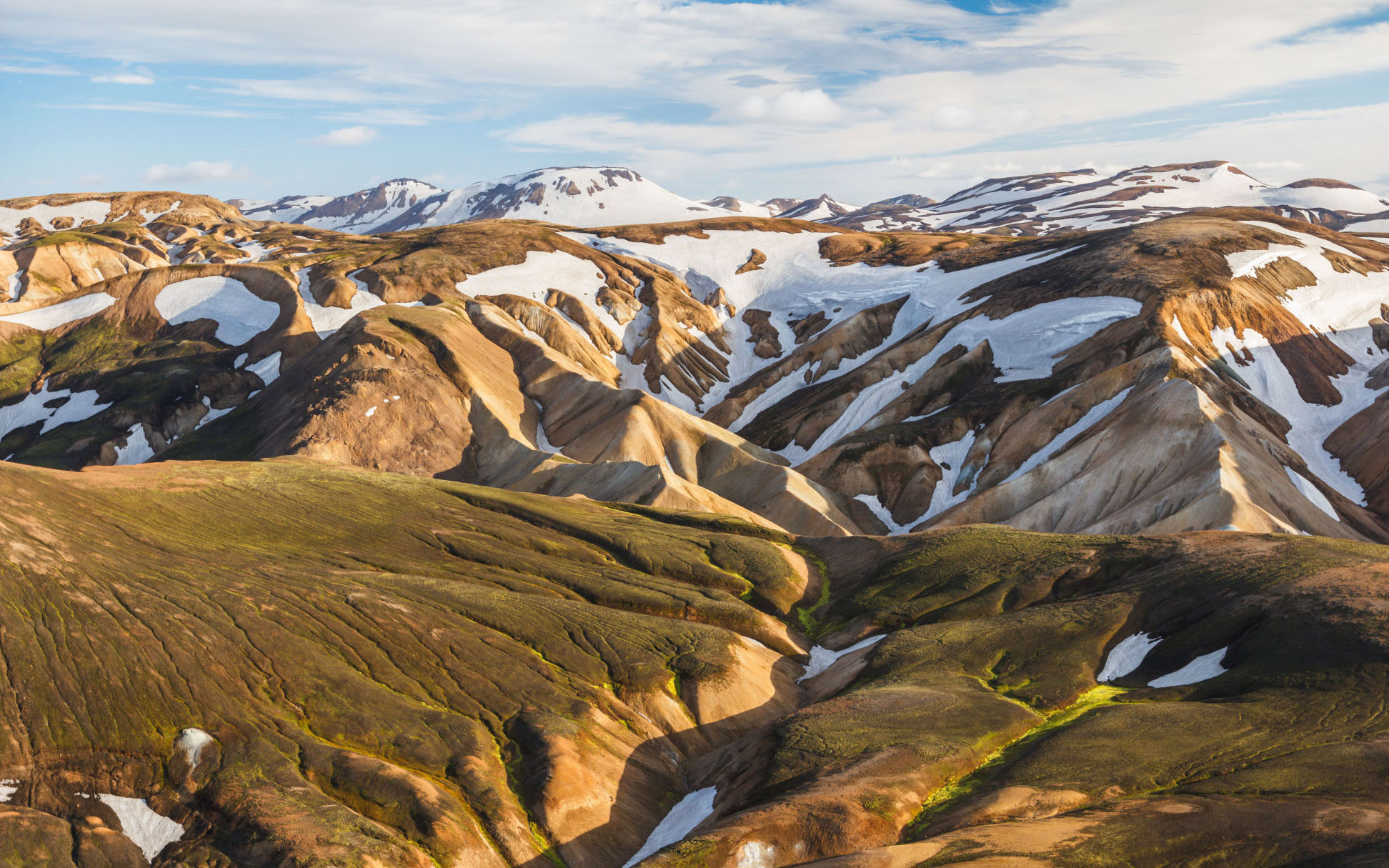
',
- '
',
+ '
',
+ '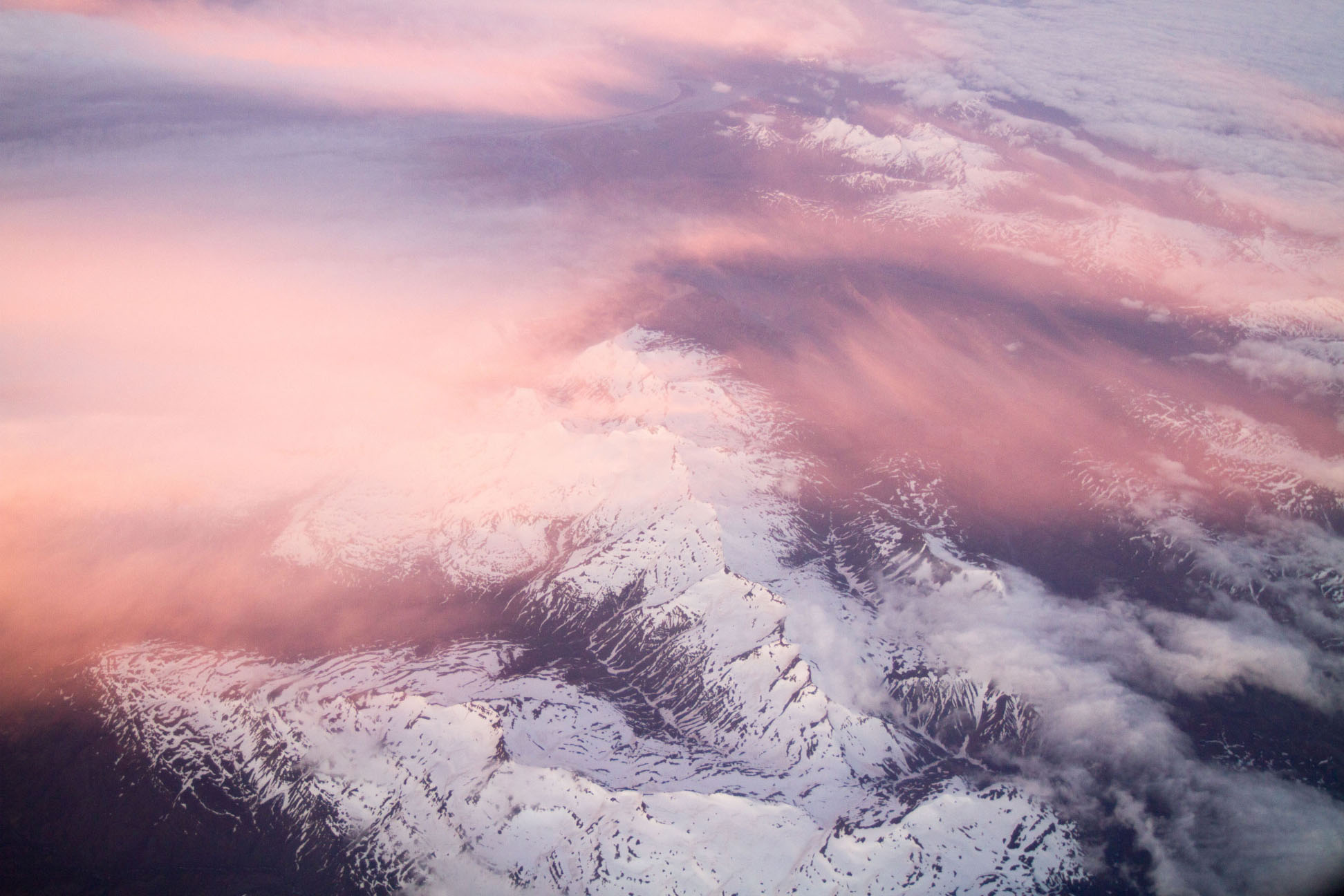
',
+ '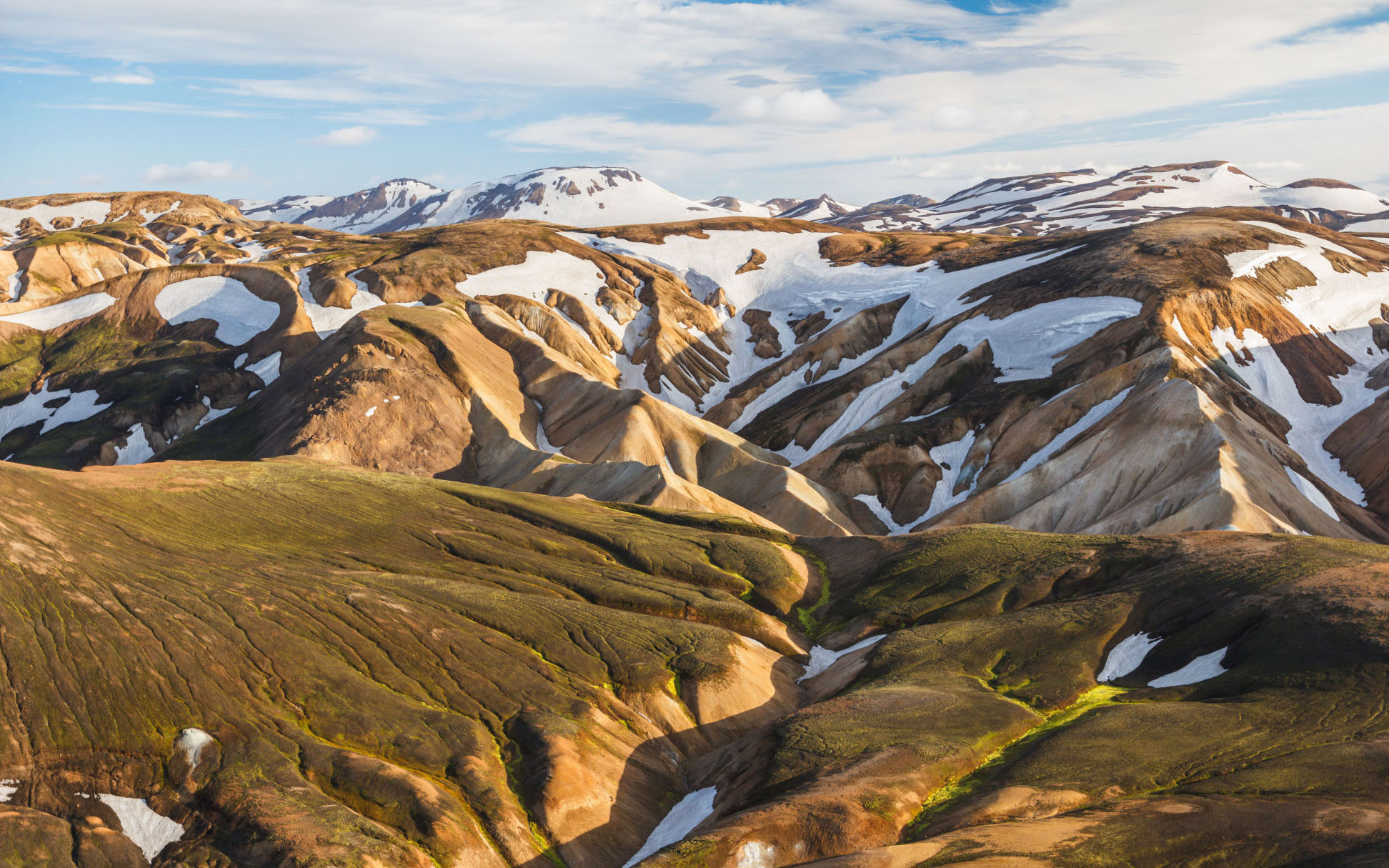
',
+ '
',
'',
'',