maxWPI) maxWPI = waypointIndex
+ //with this we get increasing amounts of filter categories for the waypoints
+ poiSite['cat'] = ["30"+(1+waypointIndex)];
+ otherSite['cat'] = ["30"+(1+waypointIndex)];
+ poiSite['cat'].push("299")
+ otherSite['cat'].push("299")
+ if (hds[systemName].hostile == "Y")
+ {
+ poiSite['cat'].push("300")
+ otherSite['cat'].push("300")
+ }
+ //console.log("adding poi with data:", poiSite, hds[systemName])
+ // We can then push the site to the object that stores all systems
+ canonnEd3d_challenge.systemsData.systems.push(poiSite);
+ canonnEd3d_challenge.systemsData.systems.push(otherSite);
+ canonnEd3d_challenge.addRoute(poiSite.cat, [poiSite.name, other.system])
+ }
+
+ //console.log("global waypoints list:", sites.wps)
+ for (var i = 0; i <= maxWPI; i++) {
+ canonnEd3d_challenge.systemsData.categories["Hyperdictions"]["30"+(1+i)] = {
+ 'name': "UIA "+(i+1),
+ 'color': '999900'
+ }
+ }
+ resolvePromise();
+ },
+ addRoute: (cat, systems, circle=false) => {
+ var route = {
+ cat: cat,
+ circle: circle,
+ points: []
+ }
+ for (var i = 0; i < systems.length; i++) {
+ route['points'].push({ 's': systems[i], 'label': systems[i] })
+ }
+ canonnEd3d_challenge.systemsData.routes.push(route);
+ },
+ uia: [],
+ formatWaypoints: function (data) {
+ var arrivaldate;
+ var arrivalcoords;
+ var arrivalname;
+ var lastarrivaldate;
+ var lastcoords;
+ var lastname;
+ var route = {
+ cat: ["101"],
+ circle: false,
+ points: []
+ }
+ var fakeroute = {
+ cat: ["103"],
+ circle: false,
+ points: []
+ }
+ var estimateroute = {
+ cat: ["102"],
+ circle: false,
+ points: []
+ }
+
+
+ var firstwp;
+ var midptime = false;
+ var lastcase = "X";
+ var lastdata = false;
+ var sumX = 0, sumY = 0, sumZ = 0;
+ var destination_reached = false;
+ // this is assuming data is an array []
+ for (var i = 0; i < data.length; i++) {
+
+ if (data[i]["System"] && data[i]["System"].replace(' ', '').length > 1) {
+
+ //manage routes
+ var curcase = data[i]["Estimate"];
+ switch (curcase){
+ //normal route points
+ case "N":
+ poicat = "101"
+ if (lastcase != "N" && lastdata) {
+ //end last route, start new
+ if (route.points.length>1) canonnEd3d_challenge.systemsData.routes.push(route);
+ route = { cat: ["101"], circle: false, points: [] }
+ //connect previous routes to this point
+ //normal routes are exclusive to surroundings, other routes are inclusive
+ if (lastcase == "F") {
+ fakeroute.points.push({s:data[i]["System"]})
+ }
+ if (lastcase == "Y") {
+ estimateroute.points.push({s:data[i]["System"]})
+ }
+ }
+ route.points.push({s:data[i]["System"]})
+ break;
+
+ //fake route points for weird behavior
+ case "F":
+ if (lastcase != "F" && lastdata) {
+ //end last route, start new
+ if (fakeroute.points.length>1) canonnEd3d_challenge.systemsData.routes.push(fakeroute);
+ fakeroute = { cat: ["103"], circle: false, points: [] }
+ //connect previous point to this route, inclusive
+ fakeroute.points.push({s:lastdata["System"]})
+ }
+ fakeroute.points.push({s:data[i]["System"]})
+ poicat = "103"
+ break;
+
+ //estimated route points
+ case "Y":
+ if (lastcase != "Y" && lastdata) {
+ //end last route, start new
+ if (estimateroute.points.length>1) canonnEd3d_challenge.systemsData.routes.push(estimateroute);
+ estimateroute = { cat: ["102"], circle: false, points: [] }
+ //connect previous fake route to this point
+ if (lastcase == "F") {
+ fakeroute.points.push({s:data[i]["System"]})
+ } else {
+ //handle the rest inclusively as estimate
+ estimateroute.points.push({s:lastdata["System"]})
+ }
+ }
+ estimateroute.points.push({s:data[i]["System"]})
+ poicat = "102"
+ break;
+ }
+ lastcase = curcase;
+ lastdata = data[i]
+
+
+ //add POIs
+ var poiSite = {};
+ poiSite['name'] = data[i]["System"];
+
+ poiSite['infos'] = '
EDSM
Signals';
+
+ //poiSite['url'] = "https://canonn-science.github.io/canonn-signals/?system=" + poiSite['name']
+ poiSite['coords'] = {
+ x: parseFloat(data[i]["X"]),
+ y: parseFloat(data[i]["Y"]),
+ z: parseFloat(data[i]["Z"]),
+ };
+ if (lastcoords) {
+ sumX += poiSite['coords'].x - lastcoords.x
+ sumY += poiSite['coords'].y - lastcoords.y
+ sumZ += poiSite['coords'].z - lastcoords.z
+ }
+
+ //Check Site Type and match categories
+ poiSite['cat'] = [poicat]
+ canonnEd3d_challenge.systemsData.systems.push(poiSite);
+
+ if (i==0) firstwp = poiSite
+
+
+
+ //manage times for the UIA
+ lastcoords = arrivalcoords
+ lastname = arrivalname
+ arrivalcoords = poiSite['coords']
+ arrivalname = poiSite['name']
+ var at = data[i]["Traversal Time"]
+ var mp = data[i]["Midpoint Time"]
+ var thetime = false;
+ midptime = false
+ if (at.indexOf("/") > 0 && at.indexOf(":") > 0) thetime = at;
+ else if (mp.indexOf("/") > 0 && mp.indexOf(":") > 0) {
+ thetime = mp
+ midptime = true
+ }
+ if (thetime) {
+ lastarrivaldate = arrivaldate
+ arrivaldate = new Date(gsheetToZuluTimestamp(thetime)).getTime()
+ }
+ }
+ if (data[i]["Remarks"].search("Destination Reached") >= 0) {
+ destination_reached = true;
+ }
+ }
+
+ if (fakeroute.points.length>1) canonnEd3d_challenge.systemsData.routes.push(fakeroute);
+ if (route.points.length>1) canonnEd3d_challenge.systemsData.routes.push(route);
+ if (estimateroute.points.length>1) canonnEd3d_challenge.systemsData.routes.push(estimateroute);
+
+ //calculating UIA current estimated position
+ if (lastcoords && lastarrivaldate) {
+ //console.log(lastcoords, lastarrivaldate, arrivalcoords, arrivaldate)
+ const start = new THREE.Vector3(lastcoords.x, lastcoords.y, lastcoords.z)
+ const end = new THREE.Vector3(arrivalcoords.x, arrivalcoords.y, arrivalcoords.z)
+ const starttime = new Date(lastarrivaldate).getTime()
+ const endtime = new Date(arrivaldate).getTime()
+ const nowtime = new Date().getTime()
+ const timediff = endtime-starttime || 1
+ const nowdiff = nowtime-starttime
+ var percent = nowdiff/timediff
+ if (midptime) percent = percent/2;
+ if (destination_reached && percent>1) percent=1;
+ const vecdiff = end.clone().sub(start)
+ canonnEd3d_challenge.uia.push(start.clone().addScaledVector(vecdiff, percent))
+ console.log("% of the way:", percent, new Date(lastarrivaldate).toString(), new Date(arrivaldate).toString())
+ var lastuia = canonnEd3d_challenge.uia.length-1
+ console.log("current estimated position of the UIA"+(lastuia+1)+": ", percent, canonnEd3d_challenge.uia[lastuia])
+ var uia_poi = {
+ 'name': "Unidentified Interstellar Anomaly "+(lastuia+1),
+ 'infos': "This is an estimate of this UIA's current position.",
+ 'url': "",
+ 'coords': {
+ x: canonnEd3d_challenge.uia[lastuia].x,
+ y: canonnEd3d_challenge.uia[lastuia].y,
+ z: canonnEd3d_challenge.uia[lastuia].z
+ },
+ 'cat': ["100"]
+ }
+ //see finishMap() for the sprite
+ canonnEd3d_challenge.systemsData.systems.push(uia_poi)
+
+ canonnEd3d_challenge.uia[lastuia].destination_reached = destination_reached;
+
+ //paint a long line of potential where the UIA is heading at
+ var meanX = sumX/data.length
+ var meanY = sumY/data.length
+ var meanZ = sumZ/data.length
+ const v3_mean = new THREE.Vector3(meanX, meanY, meanZ)
+ v3_mean.normalize()
+ var v3_firstwp = new THREE.Vector3(firstwp.coords.x, firstwp.coords.y, firstwp.coords.z)
+ v3_firstwp.addScaledVector(v3_mean, v3_firstwp.length()*predictionFactor)
+
+ //console.log(v3_mean, v3_firstwp)
+ var extension = {
+ 'name': "extended mean direction of UIA#"+(lastuia+1),
+ 'infos': "Extension system to paint the mean line of UIA#"+(lastuia+1),
+ 'url': "",
+ 'coords': { x: v3_firstwp.x, y: v3_firstwp.y, z: v3_firstwp.z },
+ 'cat': ["100"]
+ }
+ canonnEd3d_challenge.systemsData.systems.push(extension)
+ var meanroute = {
+ cat: ["100"],
+ circle: false,
+ points: [
+ {s: firstwp.name, value: firstwp.name},
+ {s: extension.name, value: extension.name}
+ ]
+ }
+ canonnEd3d_challenge.systemsData.routes.push(meanroute);
+
+
+
+ /* use once to generate poi by times and coords
+ //console.log(lastcoords, lastarrivaldate, arrivalcoords, arrivaldate)
+ const fake_start = new THREE.Vector3(-1780.65625,-592.21875,-2365.21875)
+ const fake_end = new THREE.Vector3(-1762.46875,-581.84375,-2319.875)
+ const fake_starttime = new Date(gsheetToZuluTimestamp("22/09/2022 10:05:00")).getTime()
+ const fake_endtime = new Date(gsheetToZuluTimestamp("25/09/2022 07:53:26")).getTime()
+ const fake_nowtime = new Date(gsheetToZuluTimestamp("29/09/2022 08:00:00")).getTime()
+ const fake_timediff = fake_endtime-fake_starttime || 1
+ const fake_nowdiff = fake_nowtime-fake_starttime
+ const fake_percent = fake_nowdiff/fake_timediff
+ const fake_vecdiff = fake_end.sub(fake_start)
+ //add fake lines for the UIAs in broken state//add fake lines for the UIAs in broken state
+ const fakev = fake_start.clone().addScaledVector(fake_vecdiff, fake_percent)
+ console.log(fakev)
+ //*/
+ }
+
+ },
+ formatMeasurements: async function (data, resolvePromise) {
+ //console.log(data);
+ var measystems = {};
+ for (var i = 0; i < data.length; i++) {
+ if (data[i]["Permit Lock"]) continue
+ if (data[i]["Accuracy"] > 3) continue
+ if (data[i]["Current System"]
+ && data[i]["Current System"].replace(/\s/g, '').length > 1
+ && data[i]["Targetted System"]
+ && data[i]["Targetted System"].replace(/\s/g, '').length > 1) {
+ var route = {};
+ route['points'] = [
+ { 's': data[i]["Current System"], 'label': data[i]["Current System"] },
+ { 's': data[i]["Targetted System"], 'label': data[i]["Targetted System"] }
+ ]
+ route['cat'] = ["1003"];
+ route['circle'] = false;
+ canonnEd3d_challenge.systemsData.routes.push(route);
+ if (!Object.keys(measystems).includes(data[i]["Current System"]))
+ measystems[data[i]["Current System"]] = false;
+ if (!Object.keys(measystems).includes(data[i]["Targetted System"]))
+ measystems[data[i]["Targetted System"]] = false;
+ }
+ }
+ let response = await getSystemsEDSM(Object.keys(measystems));
+
+ if (response.data.length <= 0)
+ {
+ console.log("EDSM debug", response);
+ }
+ for (const index in response.data) {
+ let system = response.data[index];
+ if (!system.name || !system.coords) continue
+ measystems[system.name] = system
+ }
+ for (let systemName in measystems) {
+ if (!measystems[systemName].name || !measystems[systemName].coords) continue;
+ var poiSite = {};
+ poiSite['name'] = measystems[systemName].name;
+ poiSite['infos'] = '
EDSM
Signals';
+ //poiSite['url'] = "https://canonn-science.github.io/canonn-signals/?system=" + poiSite['name']
+ poiSite['coords'] = {
+ x: parseFloat(measystems[systemName].coords.x),
+ y: parseFloat(measystems[systemName].coords.y),
+ z: parseFloat(measystems[systemName].coords.z),
+ };
+ //console.log(measystems[systemName])
+ poiSite['cat'] = ["1005"];
+ // We can then push the site to the object that stores all systems
+ canonnEd3d_challenge.systemsData.systems.push(poiSite);
+ }
+ resolvePromise();
+ },
+ parseCSVData: function (uri, cb, resolve) {
+ Papa.parse(uri, {
+ download: true,
+ header: true,
+ complete: function (results) {
+ return cb(results.data, resolve);
+ },
+ });
+ },
+ createSphere: function(data, color) {
+ //console.log("making sphere: ", data)
+ var geometry = new THREE.SphereGeometry(data.radius, 40, 20);
+ var sphere = new THREE.Mesh(geometry, color);
+ //idk why but the z coordinate is twisted for this
+ sphere.position.set(data.coords[0], data.coords[1], -data.coords[2]);
+ sphere.name = data.name;
+ sphere.clickable = false;
+ scene.add(sphere);
+ },
+ finishMap: function() {
+ var redmaterial = new THREE.MeshBasicMaterial({
+ color: 0xFF0000,
+ transparent: true,
+ opacity: 0.3
+ })
+ for (var i = 0; i < canonnEd3d_challenge.uia.length; i++) {
+ var sprite = new THREE.Sprite(Ed3d.material.spiral);
+ //console.log("trying stargoid sprite: ", v3_uia)
+ sprite.position.set(
+ canonnEd3d_challenge.uia[i].x,
+ canonnEd3d_challenge.uia[i].y,
+ -canonnEd3d_challenge.uia[i].z //for some reason z is inverted
+ );
+ sprite.scale.set(50, 50, 1);
+ scene.add(sprite); // this centers the glow at the mesh
+ var names = ["Taranis", "Leigong", "Indra", "Oya", "Cocijo", "Thor", "Raijin", "Hadad"]
+ if (canonnEd3d_challenge.uia[i].destination_reached) {
+ canonnEd3d_challenge.createSphere({
+ radius: 25,
+ coords: [
+ canonnEd3d_challenge.uia[i].x,
+ canonnEd3d_challenge.uia[i].y,
+ canonnEd3d_challenge.uia[i].z
+ ],
+ name: "Rogue Signal Source "+ (names[i] || (i+1))
+ }, redmaterial)
+ }
+ }
+ for (var i = 0; i < canonnEd3d_challenge.systemsData.pls.length; i++) {
+ canonnEd3d_challenge.createSphere(canonnEd3d_challenge.systemsData.pls[i], Ed3d.material.permit_zone)
+ }
+ var blackmaterial = new THREE.MeshBasicMaterial({
+ color: 0x030303,
+ transparent: true,
+ opacity: 0.3
+ })
+ for (var i = 0; i < canonnEd3d_challenge.systemsData.puls.length; i++) {
+ canonnEd3d_challenge.createSphere(canonnEd3d_challenge.systemsData.puls[i], blackmaterial)
+ }
+
+ var ygmaterial = new THREE.MeshBasicMaterial({
+ color: 0x336600,
+ transparent: true,
+ opacity: 0.3
+ })
+ for (var i = 0; i < canonnEd3d_challenge.systemsData.hd_soi.length; i++) {
+ canonnEd3d_challenge.createSphere(canonnEd3d_challenge.systemsData.hd_soi[i], ygmaterial)
+ }
+
+ var popmaterial = new THREE.MeshBasicMaterial({
+ color: 0x333300,
+ transparent: true,
+ opacity: 0.3
+ })
+ //canonnEd3d_challenge.createSphere({coords: [0,0,0], radius: 222, name: "Populated Bubble"}, popmaterial)
+
+ var gmaterial = new THREE.MeshBasicMaterial({
+ color: 0x000099,
+ transparent: true,
+ opacity: 0.15
+ })
+ for (var i = 0; i < canonnEd3d_challenge.systemsData.g_soi.length; i++) {
+ canonnEd3d_challenge.createSphere(canonnEd3d_challenge.systemsData.g_soi[i], gmaterial)
+ }
+
+ //$("#search").html("Current positions are rough estimates.
").css("display", "block").css("color", "#FF4F4F")
+
+ document.getElementById("loading").style.display = "none";
+ },
+ init: function () {
+ //var p1 = new Promise(function (resolve, reject) {
+ // canonnEd3d_challenge.parseCSVData('data/csvCache/UIA Vector Survey (Responses) - Waypoints.csv', canonnEd3d_challenge.formatWaypoints, resolve);
+ //});
+ var p2 = new Promise(function (resolve, reject) {
+ canonnEd3d_challenge.parseCSVData('data/csvCache/route_UIA_Hyperdictions.csv', canonnEd3d_challenge.formatHDs, resolve);
+ });
+
+ Promise.all([p2]).then(function () {
+ Ed3d.init({
+ container: 'edmap',
+ json: canonnEd3d_challenge.systemsData,
+ withFullscreenToggle: false,
+ withHudPanel: true,
+ hudMultipleSelect: true,
+ effectScaleSystem: [20, 500],
+ startAnim: false,
+ showGalaxyInfos: false,
+ playerPos: [0, 0, 0],
+ cameraPos: [0, 0+1000, 0-1500],
+ systemColor: '#FF9D00',
+ finished: canonnEd3d_challenge.finishMap
+ });
+ });
+ },
+};
diff --git a/data/csvCache/202011052200_Canonn Universal Science DB - TS Export - Export CSV Data.csv b/data/csvCache/202011052200_Canonn Universal Science DB - TS Export - Export CSV Data.csv
new file mode 100644
index 0000000..9e30587
--- /dev/null
+++ b/data/csvCache/202011052200_Canonn Universal Science DB - TS Export - Export CSV Data.csv
@@ -0,0 +1,230 @@
+siteID,system,planet,status,locationLat,locationLon,msg1,msg2,msg3,timesReferenced,galacticX,galacticY,galacticZ,discoveredBy
+TS1,HIP 19026,B 1 C,✘,-17.9575,-152.6994,X,X,X,1,-117.88,-65.125,-330.90625,
+TS2,ARIES DARK REGION DB-X D1-63,A 7 A,✘,25.5553,78.1765,X,X,X,1,-119.63,-90.84375,-247.03125,
+TS3,PLEIADES SECTOR OS-U C2-7,4 A,✘,58.4244,-177.0446,X,X,X,2,-68.72,-229.625,-325.6875,
+TS4,COL 285 SECTOR CV-Y D57,AB 4 A,✘,4.7654,136.2398,X,X,X,2,-21.28,-76.34375,-288.15625,
+TS5,HIP 14909,2 A,✔,-26.4561,-27.4742,TS6,TS8,TS7,4,-84.38,-259.28125,-358.65625,
+TS6,MEL 22 SECTOR ZU-P C5-1,4 A,✔,-63.5266,8.0136,TS5,TS7,TS8,4,-75.44,-284.5,-361.8125,
+TS7,MEL 22 SECTOR NX-U D2-27,5 A,✔,-39.7932,-56.254,TS9,TS8,TS6,4,-57.13,-274.71875,-373.6875,
+TS8,MEL 22 SECTOR NX-U D2-31,3 A,✔,-51.7229,-110.0287,TS7,TS6,TS5,4,-64.41,-269.5625,-373.59375,
+TS9,MEL 22 SECTOR YU-F B11-1,AB 5 A,✔,-52.0061,-5.6793,TS10,TS7,TS11,4,-33.97,-268.4375,-384.53125,
+TS10,MEL 22 SECTOR UT-R C4-4,1 A,✔,16.2444,25.5284,TS9,TS11,TS12,3,-35.56,-260.03125,-390.625,
+TS11,MEL 22 SECTOR UE-G B11-0,A 2,✔,-33.2026,141.7225,TS10,TS12,TS9,3,-39.50,-239.625,-380.625,
+TS12,HIP 16985,A 4 E,✔,40.1213,-22.6073,TS11,TS14,TS13,3,-54.59,-242.15625,-401.5,
+TS13,MEL 22 SECTOR KC-V D2-28,1 C,✔,-76.1261,113.2089,TS15,TS16,TS8,3,-78.44,-258.25,-404.0625,
+TS14,MEL 22 SECTOR BV-P C5-2,1 A,✔,-21.9701,-113.2261,TS9,TS10,TS17,2,-11.00,-270.625,-363.21875,
+TS15,MEL 22 SECTOR TT-R C4-2,3 A,✔,-27.3214,63.0988,TS12,TS16,TS13,2,-97.38,-255.21875,-419.78125,
+TS16,MEL 22 SECTOR MX-U D2-14,AB 1 A,✔,-80.3925,-101.5264,TS6,TS13,TS15,2,-107.56,-280.25,-397.5,
+TS17,MEL 22 SECTOR DG-O C6-0,2 A,✔,15.5126,-12.4966,TS14,TS19,TS18,3,5.69,-256.8125,-323.5625,
+TS18,SYNUEFE MK-M D8-20,A 2 A,✔,-73.612,-35.184,TS22,TS19,TS21,3,2.53,-266.9375,-307.40625,
+TS19,HIP 15443,AB 4 B,✔,71.5247,77.2894,TS20,TS18,TS22,3,-8.22,-259.78125,-284.78125,
+TS20,HIP 14702,1 C,✘,-8.9048,-114.7065,X,X,X,2,-30.22,-228.84375,-254.1875,
+TS21,PLEIADES SECTOR KM-W D1-40,B 10 A,✔,-35.5592,155.9887,TS30,TS3,TS39,2,-33.00,-211.9375,-327.75,
+TS22,HIP 14746,6 A,✔,76.7687,176.001,TS19,TS18,TS23,2,-22.03,-276,-290.375,
+TS23,SYNUEFE CW-V C18-6,A 3 A,✔,24.3052,-68.308,TS24,TS25,TS37,3,19.69,-223.625,-263.65625,
+TS24,SYNUEFE YP-X C17-8,2 A,✘,54.6409,-124.5742,X,X,X,2,27.38,-219,-271.4375,
+TS25,SYNUEFE OV-K D9-56,9 C,✔,-28.889,-89.2878,TS27,TS23,TS30,2,11.31,-208.5,-256.34375,
+TS26,SYNUEFE RT-Z C16-13,1 A,✔,-50.1277,44.2171,TS34,TS28,TS31,2,56.94,-142.03125,-339.9375,
+TS27,SYNUEFE YP-X C17-1,5 C,✔,48.3769,-53.768,TS24,TS25,TS37,3,32.25,-199.34375,-295.40625,
+TS28,HIP 22851,7 A,✘,-68.884,-137.8088,X,X,X,1,44.72,-106.625,-313.21875,
+TS29,PLEIADES SECTOR MC-V C2-0,1 A,✔,22.4386,121.0789,TS40,TS33,TS41,2,-5.13,-168.34375,-343.875,
+TS30,HIP 18368,2 H,✔,-21.5287,-27.1165,TS32,TS45,TS33,3,0.25,-174.8125,-286.46875,
+TS31,PLEIADES SECTOR MC-V C2-6,B 4 A,✔,25.7177,27.4909,TS29,TS33,TS34,2,6.84,-152.09375,-327.25,
+TS32,ARIES DARK REGION JW-W C1-4,7 A,✘,50.3331,-124.31,X,X,X,2,-6.22,-195.53125,-269.71875,
+TS33,PLEIADES SECTOR MC-V C2-8,8 B,✔,-31.861,-5.36,TS29,TS30,TS35,3,3.47,-159.59375,-313.84375,
+TS34,SYNUEFE JU-M D8-50,5 A,✔,-37.8668,-66.3934,TS36,TS31,TS26,2,44.69,-149,-326.84375,
+TS35,SYNUEFE UZ-X C17-12,AB 2 A,✘,39.897,-59.4823,X,X,X,1,45.41,-126.5,-288.34375,
+TS36,HIP 20486,A 3 A,✔,-33.7172,-125.1209,TS37,TS27,TS38,1,59.78,-177.34375,-300.21875,
+TS37,SYNUEFE UK-V B35-0,A 4 A,✔,-1.6378,124.6736,TS26,TS27,TS23,3,34.53,-201.53125,-290.59375,
+TS38,SYNUEFE WU-X C17-8,2 A,✘,35.4633,131.5834,X,X,X,1,30.22,-171.0625,-287.34375,
+TS39,HIP 16572,A 4 E,✘,39.1994,-29.609,X,X,X,2,-40.44,-194.5,-300.25,
+TS40,HIP 20785,6 B,✔,-39.3011,-58.6453,TS42,TS43,TS44,2,-20.75,-106.3125,-318.34375,
+TS41,SYNUEFE UZ-X C17-10,1 B,✘,31.7211,17.2641,X,X,X,1,46.06,-114.5,-293.6875,
+TS42,HIP 16440,1 E,✔,-35.0118,-157.9024,TS4,TS54,TS53,2,-74.38,-133,-267.34375,
+TS43,PLEIADES SECTOR KH-V C2-1,2 A,✘,11.4432,7.5901,X,X,X,1,-9.16,-115.78125,-307.96875,
+TS44,PLEIADES SECTOR KH-V C2-3,3 A,✔,68.9625,92.9837,TS47,TS40,TS46,2,-23.19,-115.375,-339.6875,
+TS45,HIP 19266,6 A,✔,7.8987,147.2701,TS17,TS48,TS49,2,45.25,-223.28125,-346.84375,
+TS46,PLEIADES SECTOR IR-W D1-76,B 3 A,✔,-64.438,166.8112,TS52,TS56,TS44,2,-40.81,-124.5625,-325.375,
+TS47,HIP 24007,1 B,✔,43.0054,-162.8492,TS50,TS57,TS51,2,-1.50,-52.90625,-347.46875,
+TS48,HIP 20830,A 8 F,✔,42.7052,-162.4037,TS62,TS61,TS59,3,37.78,-181.21875,-384.09375,
+TS49,MEL 22 SECTOR MC-V D2-19,BC 1 B,✔,-41.9785,-166.2575,TS45,TS17,TS55,1,31.56,-231.6875,-351.4375,
+TS50,HIP 22382,5 E,✔,16.9899,-32.5445,TS82,TS4,TS75,2,-28.91,-60.03125,-315.34375,
+TS51,SYNUEFE LD-A C17-1,B 1 C,✔,-71.1251,66.0517,TS57,TS63,TS60,3,-20.56,-61.75,-344.0625,
+TS52,DELPHI,5 A,✔,-36.5491,-143.6091,TS64,TS46,TS58,2,-63.59,-147.40625,-319.09375,
+TS53,ARIES DARK REGION MS-T C3-15,1 A,✘,-10.1076,92.2843,X,X,X,1,-57.22,-134.28125,-215.34375,
+TS54,ARIES DARK REGION FW-W D1-59,A 5 A,✘,-34.6857,-54.7454,X,X,X,1,-108.66,-128.28125,-223.03125,
+TS55,HIP 18778,A 2 A,✔,-0.6282,140.4103,TS77,TS59,TS78,3,11.47,-236.5625,-398.21875,
+TS56,COL 285 SECTOR IL-X C1-16,A 4 A,✘,-76.007,-96.1867,X,X,X,1,29.72,-76.96875,-296.3125,
+TS57,SYNUEFE LD-A C17-3,AB 2 F,✔,3.1594,24.6321,TS68,TS67,TS51,3,-23.03,-62.875,-339.375,
+TS58,PLEIADES SECTOR WE-Q B5-2,1 A,✔,49.0882,-159.7362,TS66,TS39,TS65,1,-52.59,-180.46875,-306.4375,
+TS59,HIP 20318,3 A,✔,31.254,-87.6853,TS61,TS62,TS48,3,28.38,-196.90625,-400.09375,
+TS60,HIP 25274,8 A,✘,66.3918,6.9695,X,X,X,1,18.81,-39.40625,-320,
+TS61,STRUVE'S LOST SECTOR NN-T C3-1,ABCD 1 A,✔,27.3204,134.839,TS74,TS48,TS79,4,13.53,-173.15625,-387.96875,
+TS62,STRUVE'S LOST SECTOR NN-T C3-6,1 C,✔,-53.2853,130.7533,TS74,TS59,TS61,4,8.16,-178.40625,-410.09375,
+TS63,COL 285 SECTOR IL-X C1-4,A 2 C,✘,24.0676,-175.1989,X,X,X,1,30.31,-65.21875,-294.8125,
+TS64,HIP 17694,B 7 F,✘,17.9523,-96.769,X,X,X,3,-72.25,-152.53125,-338.6875,
+TS65,PLEIADES SECTOR IR-W D1-38,ABCD 1 A,✘,-14.9126,-108.3329,X,X,X,1,-35.25,-171.8125,-324.4375,
+TS66,PLEIADES SECTOR IR-W D1-61,BC 1 A,✘,16.2778,156.3799,X,X,X,1,-50.09,-180.84375,-291.46875,
+TS67,HIP 22118,B 3 A,✔,-55.0941,155.6531,TS70,TS73,TS72,1,-7.78,-92.4375,-349.9375,
+TS68,PLEIADES SECTOR IM-V C2-16,ABC 2 A,✘,3.2208,11.7039,X,X,X,1,-9.88,-99.53125,-329.03125,
+TS69,TAURUS DARK REGION IR-W D1-47,A 3 A,✔,-29.1297,-64.9776,TS62,TS74,TS71,2,0.34,-145.03125,-422.4375,
+TS70,PLEIADES SECTOR GW-W D1-65,10 F,✘,-1.952,105.5397,X,X,X,2,-8.94,-94.6875,-343.96875,
+TS71,HIP 21251,A 8 C,✔,-4.3562,117.895,TS69,TS74,TS62,2,-2.09,-143.65625,-413.59375,
+TS72,PLEIADES SECTOR KH-V C2-7,7 F,✘,10.2451,-57.1205,X,X,X,1,3.81,-105.09375,-327.96875,
+TS73,HIP 17044,1 B,✔,-25.2169,19.5864,TS92,TS88,TS86,2,-74.25,-129.53125,-283.15625,
+TS74,TAURUS DARK REGION OX-U C2-10,4 B,✔,-8.9625,151.9945,TS71,TS69,TS61,4,11.72,-147.65625,-400.25,
+TS75,HIP 23022,B 2 A,✔,27.9425,-129.5034,TS57,TS51,TS76,2,-30.38,-51.1875,-338.15625,
+TS76,COL 285 SECTOR YO-Z C19,B 2 A,✘,46.8135,-120.6927,X,X,X,1,-48.31,-5.5,-334.09375,
+TS77,HIP 18117,5 D,✔,-27.0405,-51.5551,TS78,TS77,TS55,3,-19.50,-243.46875,-415.28125,
+TS78,STRUVE'S LOST SECTOR OI-T C3-3,2 B,✔,10.4805,1.7204,TS80,TS77,TS81,3,-32.28,-219.6875,-405.125,
+TS79,HIP 19665,8 B,✔,-15.8376,-106.2342,TS81,TS83,TS84,3,-47.13,-177.75,-457.9375,
+TS80,PLEIADES SECTOR GG-Y D33,3 A,✔,-1.0307,-155.0255,TS91,TS93,TS78,3,-37.50,-204.25,-386.3125,
+TS81,PLEIADES SECTOR DQ-Y C4,6 A,✔,-11.3492,21.792,TS80,TS79,TS85,3,-60.41,-182.3125,-420.03125,
+TS82,COL 285 SECTOR CV-Y D71,5 D,✘,-40.069,-149.5349,X,X,X,1,-45.56,-31.71875,-324.65625,
+TS83,HIP 20644,A 2 D,✔,45.9043,-46.3383,TS87,TS89,TS84,3,-50.34,-142.375,-465.125,
+TS84,TAURUS DARK REGION QN-T B3-0,1 B,✔,36.0025,136.0272,TS83,TS79,TS87,3,-40.13,-144.0625,-460.6875,
+TS85,HIP 19549,A 2 C,✔,16.8664,7.3309,TS81,TS93,TS91,3,-29.66,-169.25,-398.25,
+TS86,ARIES DARK REGION KH-V C2-4,7 A,✔,53.5298,-15.1728,TS106,TS32,TS90,1,-33.22,-180.25,-245,
+TS87,TAURUS DARK REGION BV-Y C11,8 A,✔,-34.9228,124.9054,TS83,TS84,TS94,3,-34.53,-104.71875,-468.84375,
+TS88,HIP 17837,A 4 B,✔,73.9072,176.1945,TS97,TS73,TS100,2,-52.16,-130.75,-279.9375,
+TS89,HIND SECTOR GR-W D1-13,8 H,✘,24.5778,-129.9434,X,X,X,1,-78.28,-199.59375,-473.1875,
+TS90,ARIES DARK REGION HR-W D1-34,2 A,✔,-42.187,72.608,TS95,TS121,TS122,1,-69.34,-197.6875,-253.875,
+TS91,PLEIADES SECTOR MM-V B2-2,1 B,✔,34.0179,-179.0716,TS80,TS85,TS93,3,-21.03,-179.78125,-383.75,
+TS92,HIP 11981,A 5 A,✔,-31.0007,-54.2341,TS120,TS117,TS111,1,-153.59,-123.5,-234.4375,
+TS93,HIP 18730,3 G A,✔,15.2412,-45.352,TS91,TS55,TS85,3,-24.13,-186.6875,-369.25,
+TS94,TAURUS DARK REGION CQ-Y D31,3 C,✔,-45.3895,46.9644,TS96,TS98,TS87,3,-40.94,-90.09375,-446.375,
+TS95,ARIES DARK REGION VT-R B4-0,A 6,✔,-22.6271,-15.8986,TS106,TS20,TS109,2,-61.38,-220.8125,-261.46875,
+TS96,PLEIADES SECTOR ZZ-Y C14,A 1 A,✔,13.0667,-27.9931,TS102,TS101,TS99,3,-38.19,-100.25,-411.5625,
+TS97,ARIES DARK REGION MS-T C3-2,A 1 A,✘,-4.198,-48.9098,X,X,X,1,-29.31,-138.25,-221.90625,
+TS98,HIP 22375,3 A,✔,65.9622,32.9774,TS110,TS94,TS104,3,-66.91,-66.75,-454.25,
+TS99,PLEIADES SECTOR AV-Y C7,1 B,✔,-9.4777,-95.4254,TS103,TS104,TS107,4,-65.06,-110.625,-424.3125,
+TS100,ARIES DARK REGION DB-X D1-64,4 A,✘,-17.2871,137.3867,X,X,X,1,-107.56,-99.90625,-239.25,
+TS101,PLEIADES SECTOR IX-S B4-1,10 A,✔,-2.1361,-50.3902,TS75,TS114,TS50,2,-60.78,-79.53125,-344.90625,
+TS102,TAURUS DARK REGION JH-V C2-9,AB 1 A,✔,61.2111,47.3937,TS107,TS96,TS105,3,-35.13,-77.3125,-408.3125,
+TS103,PLEIADES SECTOR EL-Y D23,ABCD 1 A,✔,-53.1279,79.7963,TS115,TS101,TS99,2,-39.63,-133.125,-410.625,
+TS104,TAURUS DARK REGION QO-Q B5-0,B 2 A,✔,-32.2019,24.7648,TS99,TS98,TS126,2,-83.75,-84.21875,-419.5625,
+TS105,HIP 22743,B 1 A,✔,16.9399,-53.3368,TS108,TS102,TS70,3,-11.34,-86.5,-399.84375,
+TS106,PLEIADES SECTOR MX-U D2-63,1 A,✘,-43.8597,85.8209,X,X,X,2,-49.84,-178.84375,-256.6875,
+TS107,TAURUS DARK REGION CQ-Y D36,7 A,✔,73.2347,168.6743,TS105,TS94,TS96,2,5.00,-94.625,-435,
+TS108,HIP 22334,1 D,✔,-54.513,-32.504,TS105,TS102,TS112,3,-24.03,-85.5,-393.78125,
+TS109,PLEIADES SECTOR MX-U D2-54,4 E,✔,-37.9907,140.6388,TS118,TS88,TS42,1,-53.34,-122.53125,-260.28125,
+TS110,HIP 21373,2 F,✔,-11.2222,-80.0124,TS113,TS98,TS119,4,-76.69,-93.03125,-460.65625,
+TS111,COL 285 SECTOR GW-W D1-66,A 3,✘,-29.0123,-153.8312,X,X,X,2,-180.13,-125.90625,-233.53125,
+TS112,HIP 21348,A 2 B,✔,50.3117,11.1931,TS115,TS108,TS114,3,-26.84,-107.90625,-379.40625,
+TS113,TAURUS DARK REGION DL-Y D54,ABC 1 C,✔,-20.9913,-31.436,TS119,TS123,TS110,4,-88.53,-124.65625,-453.40625,
+TS114,PLEIADES SECTOR DG-X C1-14,AB 1 A,✔,-32.6965,-169.7781,TS108,TS125,TS112,2,-45.63,-80.78125,-376.4375,
+TS115,HIP 20859,A 1 A,✔,32.5462,153.0528,TS112,TS103,TS116,3,-31.16,-120.65625,-384.5,
+TS116,PLEIADES SECTOR EB-X C1-2,ABC 3 C,✔,34.7054,43.2882,TS124,TS99,TS115,2,-82.66,-121.15625,-380.125,
+TS117,HIP 12967,1 A,✘,-33.6164,-95.5121,X,X,X,1,-152.56,-98.65625,-237.78125,
+TS118,ARIES DARK REGION GW-W D1-48,6 A,✘,-75.7543,-148.2469,X,X,X,1,-35.25,-124.3125,-249.5,
+TS119,HIP 20989,3 C,✔,27.8121,-69.1259,TS110,TS113,TS123,3,-78.97,-106.40625,-467.6875,
+TS120,ARIES DARK REGION JS-T C3-1,1 A,✘,-4.9532,44.6363,X,X,X,1,-166.13,-139.375,-224.875,
+TS121,HYADES SECTOR PC-V B2-3,2 A,✘,-7.0555,144.1756,X,X,X,1,13.13,-152,-221.96875,
+TS122,ARIES DARK REGION NN-T C3-12,1 D,✘,13.3934,-78.7487,X,X,X,2,-83.38,-179.125,-223.4375,
+TS123,TAURUS DARK REGION BQ-Y C12,2 A,✔,57.7906,67.3644,TS110,TS119,TS113,2,-106.59,-120.625,-477.46875,
+TS124,HIP 17862,11 E,✘,41.7995,108.2899,X,X,X,1,-81.44,-151.90625,-359.59375,
+TS125,HIP 24934,A 3 A,✘,29.6993,131.1824,X,X,X,1,-27.28,-13.84375,-319.90625,
+TS126,TAURUS DARK REGION FW-W C1-3,1 A,✔,-45.764,138.682,TS127,TS128,TS113,2,-122.34,-115.78125,-434.53125,
+TS127,MEL 22 SECTOR IS-S C4-6,ABC 2 B,✔,-46.9797,111.2209,TS134,TS133,TS131,2,-135.81,-36.375,-408.96875,
+TS128,TAURUS DARK REGION MY-R B4-1,1 B,✔,2.954,39.8759,TS130,TS129,TS132,4,-159.88,-117.53125,-427.8125,
+TS129,MEL 22 SECTOR FH-U C3-9,A 1 A,✔,39.8038,141.1577,TS128,TS130,TS132,2,-172.06,-104.4375,-446.34375,
+TS130,MEL 22 SECTOR HH-V D2-53,1 B,✔,63.2875,124.5319,TS128,TS136,TS132,3,-170.31,-126.46875,-423.0625,
+TS131,WREDGUIA VX-L D7-80,5 F,✔,68.5898,-22.5722,TS140,TS147,TS144,4,-95.38,-12.15625,-371.4375,
+TS132,TAURUS DARK REGION CL-Y D53,A 4,✔,23.2563,179.6454,TS135,TS128,TS129,4,-150.63,-143.71875,-439.625,
+TS133,PLEIADES SECTOR BG-X C1-14,5 D,✔,-51.4581,158.1264,TS142,TS138,TS145,3,-133.78,-71.96875,-373.3125,
+TS134,WREDGUIA UB-W C15-0,1 A,✔,-29.1609,148.2092,TS131,TS133,TS127,1,-142.56,-24.75,-380.90625,
+TS135,HIP 16795,A 10 F,✔,-25.8016,30.8571,TS137,TS139,TS132,3,-149.44,-167.15625,-432.125,
+TS136,MEL 22 SECTOR JX-T C3-9,6 A,✔,58.1601,53.4055,TS137,TS139,TS130,3,-183.81,-152.25,-432.5,
+TS137,MEL 22 SECTOR DB-X D1-46,A 8 A,✔,18.6182,18.6466,TS136,TS139,TS135,3,-170.31,-157.65625,-433.71875,
+TS138,MEL 22 SECTOR FM-V D2-38,A 3 A,✔,-49.2856,-159.0749,TS151,TS150,TS149,4,-172.81,-80.53125,-366.34375,
+TS139,HIP 15889,11 B,✔,-18.8713,84.8981,TS141,TS136,TS143,4,-171.53,-170.28125,-422.59375,
+TS140,TAURUS DARK REGION DB-X D1-26,6 E A,✔,-62.2121,-9.8656,TS146,TS131,TS147,2,-69.38,-22.1875,-396.125,
+TS141,HIP 16704,5 A,✔,72.5156,-130.2906,TS148,TS143,TS172,2,-118.44,-170.78125,-387.375,
+TS142,PLEIADES SECTOR BG-X C1-10,14 A,✔,52.6056,-151.842,TS126,TS133,TS151,2,-127.06,-92.90625,-384.375,
+TS143,MEL 22 SECTOR HH-V D2-37,AB 1 A,✔,16.1504,128.5936,TS139,TS135,TS137,2,-155.75,-179.3125,-406.6875,
+TS144,COL 285 SECTOR ZZ-Y D22,2 A,✘,-8.4004,31.8891,X,X,X,1,-71.94,-1.9375,-330.5625,
+TS145,PLEIADES SECTOR UZ-X B1-2,A 1 C,✘,-36.8524,101.0743,X,X,X,1,-129.13,-99.09375,-402.5625,
+TS146,WREGOE RA-W C15-18,A 1 A,✔,-13.3533,-104.0368,HIP 22460,TS147,TS47,2,-17.28,-19.0625,-379.65625,
+TS147,HIP 23269,A 1 C,✔,-48.1767,-13.3551,TS140,TS131,TS146,3,-63.69,-33,-399.3125,
+TS148,HIP 15746,AB 1 B,✔,0.8018,-144.8607,TS152,TS141,TS153,3,-143.94,-159.15625,-367.15625,
+TS149,MEL 22 SECTOR FM-V D2-67,2 A,✔,-74.1521,-82.2954,TS138,TS162,TS150,3,-202.09,-81.78125,-365.5,
+TS150,MEL 22 SECTOR NT-Q C5-11,8 A,✔,-74.7376,-152.5656,TS151,TS138,TS169,4,-174.06,-104.96875,-372.625,
+TS151,MEL 22 SECTOR ZM-H B11-3,B 3,✔,25.7074,-21.7008,TS150,TS138,TS149,3,-171.06,-92.09375,-382.75,
+TS152,PLEIADES SECTOR LN-T C3-11,9 A,✘,-72.1508,135.5127,X,X,X,1,-124.66,-113.96875,-282.625,
+TS153,PLEIADES SECTOR EC-U B3-0,4 A,✔,29.0335,153.3848,TS155,TS157,TS150,3,-166.44,-132.78125,-351.46875,
+TS154,PLEIADES SECTOR OY-R C4-19,A 3 A,✔,31.5542,141.0143,TS156,TS159,TS158,1,-73.81,-98.625,-262.3125,
+TS155,MEL 22 SECTOR OO-Q C5-6,1 A,✔,12.4032,-87.639,TS142,TS161,TS162,3,-190.78,-143.75,-354.46875,
+TS156,PLEIADES SECTOR VK-N B7-0,C 1,✘,-23.3712,-160.8387,X,X,X,1,-81.63,-100.21875,-279.375,
+TS157,PLEIADES SECTOR KT-Q B5-3,5 A,✔,0.5056,-32.957,TS1,TS153,TS160,2,-169.72,-116.6875,-322.3125,
+TS158,COL 285 SECTOR RI-R B5-3,8 B,✘,12.8468,-41.1254,X,X,X,1,-118.69,-72.28125,-239.5625,
+TS159,HYADES SECTOR AQ-Y D81,AB 3 C,✔,-53.5029,106.5668,TS165,TS167,TS168,1,-83.44,-82.78125,-244.21875,
+TS160,HYADES SECTOR XO-A C15,2 A,✘,38.6733,139.1832,X,X,X,1,-13.13,-67.875,-275.25,
+TS161,MEL 22 SECTOR LN-T D3-64,ABC 1 C,✔,-17.8016,-54.3772,TS155,TS163,TS162,3,-214.16,-136.125,-334.0625,
+TS162,MEL 22 SECTOR OO-Q C5-10,7 B,✔,4.3611,-124.1767,TS149,TS161,TS155,3,-219.69,-112.28125,-369,
+TS163,SYNUEFAI UA-Y C17-4,7 A,✔,-31.2941,-77.4125,TS164,TS161,TS166,2,-205.63,-140.9375,-296.40625,
+TS164,SYNUEFAI WV-X C17-12,2 B,✔,5.441,43.5772,TS182,TS157,TS174,3,-212.72,-177.75,-293.125,
+TS165,HYADES SECTOR ZU-Y C16,6 B,✘,-29.9773,-152.3166,X,X,X,1,-75.66,-72.625,-236.96875,
+TS166,ARIES DARK REGION HR-W D1-54,2 C A,✔,-63.0211,-76.8831,TS175,TS171,TS170,2,-143.78,-229.1875,-260.1875,
+TS167,HYADES SECTOR AQ-Y D93,2 B,✘,-46.2852,45.5708,X,X,X,1,-74.59,-70.9375,-253.96875,
+TS168,HYADES SECTOR DB-X C1-8,3 A,✘,-39.8101,36.0883,X,X,X,1,-85.75,-85.375,-219.3125,
+TS169,HIP 14756,2 E,✘,32.0728,51.8755,X,X,X,2,-159.53,-104.53125,-297.5,
+TS170,ARIES DARK REGION MX-U C2-8,A 7 A,✔,25.224,-123.7181,TS176,TS180,TS122,2,-142.00,-251.84375,-259.4375,
+TS171,ARIES DARK REGION JC-V C2-6,A 4 A,✔,-66.7152,-150.6992,TS175,TS192,TS173,4,-154.66,-222.375,-241.71875,
+TS172,PLEIADES SECTOR JC-V C2-9,1 A,✔,-13.3105,-15.3081,TS148,TS177,TS189,3,-131.41,-150.8125,-343.625,
+TS173,ARIES DARK REGION PY-R B4-1,A 4 A,✔,-44.6778,-96.0452,TS175,TS179,TS171,2,-142.88,-198.15625,-257.1875,
+TS174,SYNUEFAI YQ-X C17-8,3 A,✔,-35.3379,-127.4803,TS182,TS163,TS181,3,-192.88,-205.6875,-289.375,
+TS175,ARIES DARK REGION HR-W D1-53,7 A,✔,-52.0494,7.952,TS173,TS2,TS171,3,-140.25,-213.25,-253.0625,
+TS176,HIP 11700,1 A,✔,22.1347,96.5976,TS170,TS202,TS166,1,-114.53,-262.96875,-270.71875,
+TS177,PLEIADES SECTOR HH-V C2-6,2 A,✔,50.3267,143.9451,TS178,TS183,TS172,3,-112.31,-127.125,-318.84375,
+TS178,PLEIADES SECTOR IH-V C2-3,1 B,✔,34.0093,63.7517,TS177,TS52,TS64,1,-91.28,-114.625,-323.75,
+TS179,ARIES DARK REGION HR-W D1-44,B 2 A,✔,-18.4545,-4.6061,TS184,TS186,TS171,2,-135.72,-194.6875,-236.5625,
+TS180,ARIES DARK REGION MX-U C2-10,1 C,✔,11.4778,81.5976,TS95,TS187,TS185,1,-105.50,-235.875,-229.25,
+TS181,ARIES DARK REGION DB-X C1-2,B 1,✔,33.3162,89.9169,TS194,TS190,TS191,3,-182.78,-172.84375,-293.375,
+TS182,SYNUEFAI YQ-X C17-7,1 A,✔,-34.85,52.573,TS164,TS174,TS179,3,-209.16,-190.78125,-295.0625,
+TS183,PLEIADES SECTOR LX-U C2-2,AB 4 A,✔,8.6513,78.215,TS188,TS197,TS190,2,-134.53,-189.28125,-317,
+TS184,HIP 12916,4 A,✘,47.3301,-142.8403,X,X,X,1,-126.72,-138.625,-236.875,
+TS185,HIP 11154,B 9 B,✘,2.1539,65.5808,X,X,X,1,-104.19,-195.8125,-206.25,
+TS186,ARIES DARK REGION FW-W D1-76,4 A,✘,-61.213,0.2473,X,X,X,1,-136.75,-130.34375,-207.90625,
+TS187,ARIES DARK REGION OI-T C3-8,1 A,✘,66.8546,-149.872,X,X,X,1,-114.53,-210.25,-208.25,
+TS188,PLEIADES SECTOR JM-W D1-52,2 A,✔,-32.4659,-65.5308,TS193,TS3,TS195,2,-93.78,-198.84375,-332.5625,
+TS189,PLEIADES SECTOR HH-V C2-13,A 1 C,✔,-35.6606,-38.1952,TS172,TS177,TS153,1,-134.88,-139.375,-327.90625,
+TS190,MEL 22 SECTOR LN-T D3-60,5 A,✔,41.5369,7.7036,TS181,TS182,TS164,3,-183.53,-179.5,-314.375,
+TS191,MEL 22 SECTOR WK-O C6-1,5 C,✔,33.9557,16.7738,TS200,TS199,TS208,3,-191.91,-221.3125,-320.375,
+TS192,ARIES DARK REGION IH-V C2-9,6 B,✘,30.7249,-175.8981,X,X,X,1,-105.59,-172.65625,-233.375,
+TS193,PLEIADES SECTOR OD-S B4-1,2 A,✔,62.8175,137.8808,TS64,TS116,TS188,1,-93.41,-164,-341.59375,
+TS194,ARIES DARK REGION DB-X C1-7,A 9 A,✔,-29.4982,-169.2346,TS181,TS196,TS190,1,-168.13,-173.90625,-281.15625,
+TS195,PLEIADES SECTOR OS-U C2-6,A 5 B,✔,34.5205,-87.6166,TS21,TS5,TS198,2,-104.31,-239.25,-336.5625,
+TS196,ARIES DARK REGION AQ-Y D14,4 A,✘,-43.779,-72.0815,X,X,X,1,-159.53,-144.25,-268.90625,
+TS197,PLEIADES SECTOR JS-T C3-17,1 A,✘,-29.0631,73.5608,X,X,X,1,-122.50,-101.875,-289.75,
+TS198,MEL 22 SECTOR CB-O C6-4,3 A,✔,46.0061,-77.1934,TS195,TS5,TS199,2,-122.66,-267.5,-336.84375,
+TS199,MEL 22 SECTOR SK-E B12-1,2,✔,40.8408,-104.0889,TS198,TS203,TS201,3,-149.31,-228.09375,-351.25,
+TS200,MEL 22 SECTOR JC-V D2-41,A 1 A,✔,44.6542,39.6236,TS206,TS203,TS207,4,-189.84,-214.71875,-350.8125,
+TS201,MEL 22 SECTOR JC-V D2-15,ABC 1 A,✔,38.6711,-86.7048,TS203,TS200,TS204,3,-176.09,-203.34375,-368.65625,
+TS202,HIP 11457,1 C,✘,-34.7251,-78.8895,X,X,X,1,-118.22,-235.3125,-250.78125,
+TS203,MEL 22 SECTOR JC-V D2-14,A 4 A,✔,22.3928,-135.0533,TS183,TS204,TS200,4,-174.16,-200.96875,-361.71875,
+TS204,MEL 22 SECTOR IO-G B11-1,1 A,✔,-18.0788,42.2722,TS205,TS148,TS206,4,-198.09,-202.34375,-381.90625,
+TS205,MEL 22 SECTOR OY-R C4-5,2 A,✔,34.9502,136.1186,TS204,TS201,TS203,2,-194.38,-207,-388.65625,
+TS206,MEL 22 SECTOR JC-V D2-19,4 B,✔,9.4863,-108.1907,TS191,TS200,TS204,2,-203.19,-219.625,-356.65625,
+TS207,MEL 22 SECTOR JC-V D2-31,A 16 B,✔,40.0326,164.0504,TS201,TS199,TS205,1,-158.59,-232.78125,-392.96875,
+TS208,ARIES DARK REGION HR-W C1-1,8 C,✔,21.0004,75.8452,TS209,TS191,TS174,1,-169.47,-231.34375,-298.71875,
+TS209,MEL 22 SECTOR AX-A B14-1,1 B,✘,-8.1745,65.0438,X,X,X,1,-164.31,-237.28125,-319.78125,
+TS210,HYADES SECTOR DB-X C1-19,8 C,✘,27.1036,-128.5733,X,X,X,1,-79.94,-88.375,-219.8125,
+TS211,ARIES DARK REGION IM-V C2-5,12 A,✘,16.5321,-8.3025,X,X,X,1,-27.03,-115.21875,-262.625,
+TS212,HIP 15134,3 B,✘,-14.3252,-67.3716,X,X,X,1,-48.09,-136.65625,-198.8125,
+TS213,HIP 20964,3 B,✘,19.4297,-146.9173,X,X,X,1,-35.78,-61.375,-245.96875,
+TS214,PLEIADES SECTOR FW-W D1-37,2 A,✔,37.6546,39.9261,TS216,TS215,TS213,1,-79.69,-79.28125,-279.5625,
+TS215,HIP 18909,A 4 E A,✔,60.0704,150.5113,TS211,TS212,TS217,1,-24.56,-107.3125,-237.75,
+TS216,HIP 14908,A 2 A,✘,70.8429,19.5973,X,X,X,1,-74.84,-127.65625,-218.28125,
+TS217,ARIES DARK REGION GW-W D1-86,1 B,✘,-28.2303,99.013,X,X,X,1,-21.25,-129.75,-227.875,
+TS218,PLEIADES SECTOR LS-T C3-2,5 A,✔,10.0272,45.0325,TS214,TS219,TS210,1,-58.97,-81.5625,-296.53125,
+TS219,PLEIADES SECTOR GW-W D1-54,2 C,✔,76.9958,-139.547,TS154,TS218,TS220,1,-46.63,-91.6875,-271.03125,
+TS220,HIP 22347,4 A,✘,17.9813,140.8444,X,X,X,1,14.44,-80.15625,-259.5625,
+TS221,COL 285 SECTOR BQ-X C1-10,1 A,✘,37.1876,-149.1016,X,X,X,2,-172.03,-61.625,-272.34375,
+TS222,SYNUEFAI RK-Y C17-3,A 1 A,✔,47.3339,-16.4177,TS223,TS224,TS169,1,-180.19,-64.375,-300.6875,
+TS223,HIP 14969,CD 2 A,✔,-3.7118,89.4021,TS222,TS221,TS225,1,-193.97,-72.75,-315.09375,
+TS224,HIP 16692,4 C,✘,-20.2772,152.5539,X,X,X,1,-109.94,-61.5625,-235.8125,
+TS225,SYNUEFAI UZ-M D8-63,B 1 A,✔,-53.0775,46.9689,TS226,TS221,TS111,1,-198.56,-99.3125,-269.25,
+TS226,COL 285 SECTOR EB-X D1-39,4 A,✘,-1.2455,-139.3824,X,X,X,1,-157.78,-83.625,-260.5,
+TS227,PLEIADES SECTOR DG-X C1-11,A 3 A,✘,-3.2977,-131.8149,X,X,X,1,-49.25,-70.4375,-360.8125,
+TS228,SYNUEFE HZ-M D8-69,B 7 F,✔,6.4332,-124.9072,X,X,X,0,28.03,-89.375,-338.6875,
+TS229,HIP 22711,7 A,✔,16.3571,-135.0391,TS227,HIP 22460,X,0,-35.75,-60.84375,-366.90625,Thepirateorc
\ No newline at end of file
diff --git a/data/csvCache/Listening_Posts.csv b/data/csvCache/Listening_Posts.csv
new file mode 100644
index 0000000..e67aee3
--- /dev/null
+++ b/data/csvCache/Listening_Posts.csv
@@ -0,0 +1,130 @@
+System,link0_X,link0_Y,link0_Z,Linked system 1,link1_X,link1_Y,link1_Z,Linked system 2,link2_X,link2_Y,link2_Z,Points to system,target_X,target_Y,target_Z,Related discovery,Canonn,Other,Notes,Solved?
+42 N Persei,-83.5625,-73.40625,-244.34375,,,,,,,,,HIP 21478,-35.4375,-63.625,-280.125,Survey Vessel Pandora,https://canonn.science/codex/survey-vessel-pandora/,,,yes
+5 Chi Lupi,61.3125,51.40625,178.0,Ruka,30.71875,42.03125,163.375,Scorpii Sector IR-W c1-35,61.15625,13.34375,155.84375,HR 5991,56.09375,33.21875,166.375,Research Facility 5592,https://canonn.science/codex/research-facility-5592/,,,yes
+58 Eridani,20.78125,-24.40625,-29.15625,,,,,,,,,LP 892-51,45.875,-32.21875,-33.40625,Megaship Nemb,https://canonn.science/codex/nemb/,,,yes
+Achenar,67.5,-119.46875,24.84375,,,,,,,,,,,,,Pilots' Memorial: Achenar,,https://forums.frontier.co.uk/threads/elite-dangerous-in-game-memorials.566260/,,yes
+Aiabiko,-82.0625,1.625,-14.4375,,,,,,,,,,,,,Joy Senne Ice Beacon,,https://community.elitedangerous.com/galnet/30-SEP-3308,"Scanning the LP will give 3 audio messages and unlock a xeno-affirmative ship nameplate set and decal, as mentioned in a Galnet post.",yes
+Alacarakmo,-32.40625,169.53125,-49.4375,,,,,,,,,,,,,Distress Call: Diamond Bear,,https://forums.frontier.co.uk/threads/buckyball-racing-club.193467/page-131#post-8634982,"Diamond Bear Log 1/1 - Related to the Buckyball Racing Club, supposedly part of a series of LPs but no other LPs have been found.",probably
+Alioth,-33.65625,72.46875,-20.65625,,,,,,,,,,,,,Pilots' Memorial: Alioth,,https://forums.frontier.co.uk/threads/elite-dangerous-in-game-memorials.566260/,,yes
+Alpha Centauri,3.03125,-0.09375,3.15625,George Pantazis,-12.09375,-16.0,-14.21875,,,,,Wise 0855-0714,6.53125,-2.15625,2.03125,,https://canonn.science/codex/unsolved-alvin-deefer/,,Mystery of Alvin Deefer and his ship the Shady Lady,no
+Alrai Sector WZ-P b5-6,-38.625,-3.25,122.21875,,,,,,,,,Ross 859,-31.8125,15.9375,162.125,Generation Ship Odysseus,https://canonn.science/codex/generation-ship-odysseus/,,,yes
+Asellus Primus,-23.9375,40.875,-1.34375,,,,,,,,,,,,,Pilots' Memorial: Asellus Primus,,https://forums.frontier.co.uk/threads/elite-dangerous-in-game-memorials.566260/,,yes
+Batar,97.78125,99.34375,-81.65625,Chipi,119.03125,95.46875,-62.59375,Waikiri,92.75,136.5,-23.90625,Koli Discii,108.84375,83.53125,-58.71875,Koli Discii Crashed Ship,https://canonn.science/codex/koli-discii-crashed-ship/,,Latitude and longitude appear to be inverted and the star “C” is missing in the messages,yes
+Belispel,-120.59375,-1.5,-57.875,Col 285 Sector GI-H b11-6,-171.75,-5.40625,-115.09375,Pegasi Sector TT-X a2-2,-142.625,-42.21875,-94.4375,Col 285 Sector UZ-O c6-9,-144.21875,-12.25,-87.71875,Orion’s Folly,https://canonn.science/codex/orions-folly/,,,yes
+Bhadaba,-18.96875,-19.40625,-49.78125,HIP 16378,-118.75,-155.375,-354.875,,,,,HIP 16378,-118.75,-155.375,-354.875,Survey Vessel Stargazer,https://canonn.science/codex/survey-vessel-stargazer/,,Crashed ship logs further points to Thargoid Structure on HIP 17694 B 7 f,yes
+Bilfrost,-10.28125,100.90625,-0.65625,,,,,,,,,Virudnir,-12.78125,143.28125,-66.125,Generation Ship Lazarus Expedition,https://canonn.science/codex/lazarus-expedition/,,,yes
+Celaeno,-81.09375,-148.3125,-337.09375,,,,,,,,,Electra,-86.0625,-159.9375,-361.65625,Hogan Class Bulk Cargo Ship PTK-179,https://canonn.science/codex/hogan-class-bulk-cargo-ship-ptk-179/,,"LP msg used to link to HIP 17862, but has been changed to Electra. Ship details do not match msg, ship link doesn't give a msg.",probably
+Cerritics,1.0,43.03125,-163.53125,Hun Nu,-76.03125,50.1875,-110.28125,,,,,HIP 32001,-59.90625,58.78125,-125.96875,Ariane’s Pride,https://canonn.science/codex/arianes-pride/,https://forums.frontier.co.uk/threads/new-listening-post-and-crash-site.466925/,Points to crashed T9 found in HIP 32001 3 a,yes
+Col 285 Sector AV-M b21-5,138.84375,-131.4375,98.65625,,,,,,,,,Coelachi,178.25,-173.6875,109.8125,Generation Ship Phobos,https://canonn.science/codex/generation-ship-phobos/,,,yes
+Col 285 Sector AX-S a33-0,-43.8125,7.21875,327.46196,Col 285 Sector SF-N c7-2,-12.1875,-35.34375,350.53067,Col 285 Sector UB-T a33-4,-27.5625,13.15625,364.96764,Col 285 Sector NP-W a31-3,-347.5,-27.8125,-5.625,Extraction Site HS-98,https://canonn.science/codex/extraction-site-hs-98/,https://www.youtube.com/watch?v=nA7gsZexgeI,,yes
+Col 285 Sector AZ-B b15-0,-263.28125,165.5,-27.75,Col 285 Sector PH-E b14-2,-283.28125,217.3125,-46.78125,HR 6348,-267.53125,199.6875,0.21875,Aldhibah,-267.53125,188.5625,-27.8125,Lookout Military Test Facility,https://canonn.science/codex/lookout-military-test-facility/,,,yes
+Col 285 Sector CI-V b18-0,55.375,198.53125,40.4375,HIP 63386,84.71875,288.25,71.5,Mel 111 Sector BL-O b6-0,54.5625,282.40625,5.46875,34 Virginis,61.75,261.75,37.65625,Abandoned SRV,https://canonn.science/codex/34-virginis-abandoned-srv/,,,yes
+Col 285 Sector DY-F b25-0,-78.28125,-115.6875,187.46875,Col 285 Sector YF-O d6-99,-172.03125,-101.21875,142.0,HIP 97380,-154.625,-46.78125,303.75,Alshat,-119.375,-111.125,193.71875,Expedition Vessel Odysseus,https://canonn.science/codex/expedition-vessel-odysseus/,,"Points to a derelict Anaconda, an SRV is 247km away and Clarke's Rest is 162km away.",yes
+Col 285 Sector EC-J c10-4,119.9375,237.40625,67.03125,Col 285 Sector IZ-R b20-2,63.59375,227.6875,82.75,Col 285 Sector KD-H c11-3,124.1875,175.15625,101.125,44 k Virginis,103.9375,217.15625,81.5625,Abandoned SRV,https://canonn.science/codex/44-k-virginis-abandoned-srv/,,,yes
+Col 285 Sector EC-U d3-59,-305.09375,186.59375,-73.65625,Col 285 Sector EF-A b16-1,-260.59375,155.15625,-21.15625,Col 285 Sector LO-P c6-1,-251.875,119.15625,-90.5,Col 285 Sector MZ-N c7-13,-276.5625,155.90625,-57.78125,Botanical Research Station FNA-559,https://canonn.science/codex/botanical-research-station-fna-559/,https://imgur.com/a/Ijv3wc5,,yes
+Col 285 Sector ER-V d2-46,-213.8125,81.9375,-127.21875,HIP 12959,-245.84375,83.4375,-208.8125,Col 285 Sector GM-V d2-72,-221.53125,20.53125,-159.15625,Col 285 Sector OP-L b9-0,-224.875,60.375,-163.875,Unnamed Crash Site,https://canonn.science/codex/col-285-sector-op-l-b9-0-unnamed-crash-site/,,The crash site seems to have been removed,yes
+Col 285 Sector FG-X d1-49,90.15625,40.75,-211.375,Hyades Sector EB-X d1-59,78.59375,45.0,-153.875,Hyades Sector IR-U b3-5,73.9375,-16.96875,-185.96875,HR 2551,88.71875,16.59375,-182.09375,Dixon Dock,https://canonn.science/codex/dixon-dock/,,,yes
+Col 285 Sector FW-D c12-24,147.90625,-107.0625,155.3125,Synuefe DP-J b56-5,196.78125,-96.1875,153.84375,Synuefe KM-E b59-10,143.96875,-83.78125,210.0,Synuefe JB-G b58-6,168.125,-98.125,177.3125,Exploration Camp C-NO4,https://canonn.science/codex/exploration-camp-c-no4/,,,yes
+Col 285 Sector GN-H b11-0,-142.09375,-1.09375,-122.15625,Col 285 Sector MY-O b7-2,-128.78125,35.6875,-186.9375,Pic Tok,-81.46875,-22.875,-160.84375,Hyades Sector DR-V c2-23,-104.625,-0.8125,-151.90625,Dav’s Hope,https://canonn.science/codex/davs-hope/,,,yes
+Col 285 Sector HG-F b27-0,-80.5625,179.1875,220.71875,Col 285 Sector JJ-F c12-10,-66.84375,180.71875,161.75,HIP 79256,-104.09375,185.375,174.78125,Col 285 Sector ZT-I b25-0,-80.84375,180.46875,181.53125,Planet Dave Outpost,https://canonn.science/codex/planet-dave-outpost/,,,yes
+Col 285 Sector KL-Y b16-2,-215.6875,160.84375,-1.59375,Col 285 Sector SR-F b13-1,-213.46875,180.96875,-72.0625,HIP 73773,-126.625,138.5625,-28.4375,Col 285 Sector OZ-N c7-13,-189.21875,159.875,-34.84375,Exploration Camp JSPR-003,https://canonn.science/codex/exploration-camp-jspr-003/,,,yes
+Col 285 Sector KM-V b18-3,-249.53125,227.75,48.34375,HR 6514,-326.5,218.9375,16.21875,Wredguia IT-J b51-2,-333.84375,262.90625,43.9375,HIP 83237,-305.21875,235.3125,34.15625,Site 16,https://canonn.science/codex/site-16/,,,yes
+Col 285 Sector KO-E b26-3,73.34375,-84.59375,196.78125,HIP 97146,15.75,-91.3125,168.125,Pulano,58.21875,-75.90625,140.0,Col 285 Sector BG-O d6-93,45.78125,-83.84375,161.90625,Communication Array Delta 69,https://canonn.science/codex/communication-array-delta-69/,,,yes
+Col 285 Sector LJ-P c6-17,-306.0625,73.6875,-83.71875,Col 285 Sector ML-Z b15-5,-256.0,79.75,-23.53125,HIP 98328,-348.34375,80.78125,6.1875,Alshat,-119.375,-111.125,193.71875,SRV-Odysseus,https://canonn.science/codex/srv-odysseus/,,Related to nearby Expedition Vessel Odysseus which has its own set of LPs,yes
+Col 285 Sector LU-M c8-6,-249.0,264.25,1.8125,Col 285 Sector QA-L c9-3,-220.8125,263.4375,35.21875,Col 285 Sector RV-K c9-8,-242.59375,227.1875,32.6875,Col 285 Sector FL-X b17-3,-238.6875,253.875,20.15625,Medical Research Base MIR-14,https://canonn.science/codex/medical-research-base-mir-14/,,,yes
+Col 285 Sector NO-Q d5-59,-256.03125,151.59375,125.375,Col 359 Sector QC-B c1-11,-317.21875,177.71875,148.375,Col 359 Sector QI-Z c1-11,-502.0625,182.71875,190.0625,HIP 86908,-273.71875,164.6875,150.1875,Quarantine Site UC-001,https://canonn.science/codex/quarantine-site-uc-001/,,,yes
+Col 285 Sector NV-N b7-4,168.0625,-73.90625,-192,,,,,,,,,Synuefe FM-S B37-4,208.71875,-138.84375,-255.4375,Hesperus beacon TX-B-03,https://canonn.science/codex/proteus/,https://inara.cz/galaxy-logbooks/61035/,"Trail of beacons left behind on the path of the Hesperus, see inara link for an interesting logbook.",yes
+Col 285 Sector OR-F b26-2,-139.46875,41.5625,200.21875,Col 285 Sector YC-F b26-9,-55.875,-14.0,203.28125,Temnet,-83.0625,35.0,116.46875,HR 6890,-91.3125,13.625,179.625,Transmitter VJS-81,https://canonn.science/codex/transmitter-vjs-81/,,,yes
+Col 285 Sector WH-O a22-2,143.75,-33.5625,-106.53125,,,,,,,,,Col 285 Sector NV-N b7-4,168.0625,-73.90625,-192,Hesperus beacon TX-B-02,https://canonn.science/codex/proteus/,https://inara.cz/galaxy-logbooks/61035/,"Trail of beacons left behind on the path of the Hesperus, see inara link for an interesting logbook.",yes
+Col 359 Sector HT-H b40-2,27.40625,188.65625,979.875,Col 359 Sector NZ-F b41-1,60.8125,185.90625,1014.28125,Col 359 Sector UE-G d11-8,-17.21875,179.3125,1054.5,Col 359 Sector RY-H d10-58,19.53125,177.90625,1013.5,Exploration Camp JSPR-003 (#2),https://canonn.science/codex/exploration-camp-jspr-003-2/,,"This camp has the same name, layout, and backstory as the one on Col 285 Sector OZ-N c7-13 BC 3",yes
+Col 359 Sector KB-W D2-47,0.65625,149.78125,306.5625,Col 359 Sector TV-J B11-4,19.0625,123.375,371.09375,Praea Euq NN-S b4-0,80.125,115.96875,320.875,HR 6051,34.03125,124.53125,330.15625,Trading Vessel MD-002,https://canonn.science/codex/trading-vessel-md-002/,,,yes
+Col 359 Sector LH-J b10-5,-542.21875,-114.59375,345.25,Col 359 Sector NH-J b10-1,-496.5,-119.25,339.1875,Col 359 Sector RN-S c4-14,-526.78125,-105.53125,300.59375,Col 359 Sector RN-S c4-12,-519.59375,-111.40625,327.78125,Medical Research Base BJI-86,https://canonn.science/codex/medical-research-base-bji-86/,,,yes
+Col 69 Sector JI-I c10-4,439.40625,-237.59375,-1208.71875,,,,,,,,,Wregoe DK-R b4-1,171.65625,7.5625,-951.1875,Commander Hyford storyline (2/4),https://canonn.science/codex/colonia-crash-site/,https://www.youtube.com/watch?v=hisbQoaDox8,"Series of LPs, but story begins at ""Commander Hyford storyline (start)"" in Colonia",yes
+Col 69 Sector LY-H c10-0,359.75,-325.03125,-1185.3125,,,,,,,,,Trapezium Sector YU-X c1-2,573.59375,-339.46875,-1167.65625,"Hesperus beacon TX-B-17, Proteus",https://canonn.science/codex/proteus/,https://inara.cz/galaxy-logbooks/61035/,Final beacon left behind by the Hesperus crew that escaped in the Proteus,yes
+Col 69 Sector VK-E c12-10,440.84375,-309.40625,-1136.9375,,,,,,,,,Col 69 Sector LY-H c10-0,359.75,-325.03125,-1185.3125,Hesperus beacon TX-B-16,https://canonn.science/codex/proteus/,https://inara.cz/galaxy-logbooks/61035/,"Trail of beacons left behind on the path of the Hesperus, see inara link for an interesting logbook.",yes
+Colonia,-9530.5,-910.28125,19808.125,,,,,,,,,,,,,Pilots' Memorial: Colonia,,https://forums.frontier.co.uk/threads/elite-dangerous-in-game-memorials.566260/,,yes
+Colonia,-9530.5,-910.28125,19808.125,Wasat,16.3125,17.125,-55.65625,Neche,63.6875,12.90625,-13.09375,Colonia,-9530.5,-910.28125,19808.125,Commander Hyford storyline (start),https://canonn.science/codex/colonia-crash-site/,https://www.youtube.com/watch?v=WJI0J4sOxS8&t=591s,,yes
+Colonia,-9530.5,-910.28125,19808.125,,,,,,,,,,,,,Memorial for CMDR Garak,,,,yes
+Delphi,-63.59375,-147.40625,-319.09375,HIP 16753,-88.3125,-172.9375,-348.84375,Pleiades Sector OI-T c3-7,-69.4375,-180.4375,-287.625,HIP 16613,-63.9375,-186.6875,-325.4375,"The Bug Killer (Crashed Anaconda, SRV)",https://canonn.science/codex/bug-killer-crashed-anaconda/,,"System ""Pleiades Sector IR-W d1-55"" was renamed to Delphi",yes
+Dromi,25.40625,-31.0625,41.625,,,,,,,,,,,,,Pilots' Memorial: Dromi,,https://forums.frontier.co.uk/threads/elite-dangerous-in-game-memorials.566260/,,yes
+EE Leonis,11.28125,18.09375,-5.5625,,,,,,,,,Charick Drift,8.1875,26.71875,-6.0625,Generation Ship Atlas,https://canonn.science/codex/generation-ship-atlas/,,,yes
+Electra,-86.0625,-159.9375,-361.65625,Pleiades Sector KC-V c2-4,-88.8125,-148.71875,-321.1875,Pleiades Sector PD-S b4-0,-80.625,-156.6875,-337.4375,HIP 16217,-107.84375,-155.5625,-333.28125,Penal Colony BV-2259,https://canonn.science/codex/penal-colony-bv-2259/,,,yes
+Evelyn`s Light,-9236.0625,-1326.03125,19330.03125,,,,,,,,,,,,,Memorial for CMDR Evelyn Faye Roy,,,,yes
+Flame Sector PI-T b3-0,378.59375,-340.6875,-882.15625,,,,,,,,,NGC 1999 Sector HN-S B4-0,455.625,-343.8125,-941.4375,Hesperus beacon TX-B-12,https://canonn.science/codex/proteus/,https://inara.cz/galaxy-logbooks/61035/,"Trail of beacons left behind on the path of the Hesperus, see inara link for an interesting logbook.",yes
+Gliese 452.3,30.0,127.6875,-15.5,HR 4535,58.4375,195.59375,-22.6875,Mel 111 Sector CL-P b5-1,-20.25,208.96875,-13.8125,Col 285 Sector YF-M c8-8,26.8125,162.78125,-20.1875,Jackson Enterprise,https://canonn.science/codex/jackson-enterprise/,,,yes
+Graffias,41.46875,157.96875,369.6875,Lupus Dark Region JY-Q b5-1,117.1875,139.6875,357.09375,Lupus Dark Region DW-V b2-1,78.65625,177.90625,423.46875,HR 5906,81.65625,154.53125,382.21875,Unregistered Derelict (Anaconda),https://canonn.science/codex/unregistered-derelict/,,,yes
+Halbangaay,-51.9375,-95.625,10.0625,,,,,,,,,Kachirigin,-105.375,-73.34375,27.75,Megaship Welcome Deviation,https://canonn.science/codex/the-welcome-deviation-story/,,Megaship messages further lead to Scientific Installation Relay Station JE-6060 near Guara 4,yes
+HD 63587,23.0625,481.875,-894.25,HIP 37105,-10.21875,469.34375,-960.84375,Wregoe RZ-W c2-1,85.875,479.1875,-930.65625,Wregoe TJ-Z d6,27.96875,479.40625,-928.09375,Colony Site BNI-87,https://canonn.science/codex/colony-site-bni-87/,https://imgur.com/a/m2Yj8az,,yes
+Heart Sector EB-X c1-12,-5313.03125,164.8125,-5282.1875,,,,,,,,,,,,,Memorial for CMDR Alex McKinnon,,https://twitter.com/elitedangerous/status/1297164141304651776,,yes
+Heart Sector IR-V b2-0,-5303.78125,130.34375,-5305.40625,,,,,,,,,,,,,Memorial for CMDR Fluk,,,,yes
+HIP 105408,18.15625,-147.71875,145.5,,,,,,,,,HIP 106288,15.5625,-158.0625,145.78125,Janus Corp. Medical Research Centre,https://canonn.science/codex/janus-corp-medical-research-centre/,,,yes
+HIP 15787,-134.125,-116.21875,-308.28125,Pleiades Sector IH-V c2-16,-92.0,-143.5,-341.3125,Pleiades Sector AB-W b2-4,-137.5625,-118.25,-380.4375,Pleiades Sector JN-S b4-3,-112.78125,-109.03125,-333.71875,Scrump Landing,https://canonn.science/codex/scrump-landing/,,,yes
+HIP 16431,-109.15625,-159.5,-348.21875,Taygeta,-88.5,-159.6875,-366.28125,Pleiades Sector DL-Y d40,-99.15625,-146.53125,-382.09375,HIP 17403,-93.6875,-158.96875,-367.625,Research Base LV 87,https://canonn.science/codex/research-base-lv-87/,,,yes
+HIP 17044,-74.25,-129.53125,-283.15625,Pleiades Sector IH-V c2-5,-78.71875,-138.03125,-321.78125,Pleiades Sector HR-W d1-57,-86.40625,-125.15625,-301.84375,Pleiades Sector HR-W d1-17,-84.875,-123.8125,-315.25,Research Base KG-3362,https://canonn.science/codex/research-base-kg-3362/,,,yes
+HIP 17225,-86.15625,-165.59375,-356.8125,Pleiades Sector IH-V c2-16,-92.0,-143.5,-341.3125,,,,,Celaeno,-81.09375,-148.3125,-337.09375,Relay Station PSJ-17,https://canonn.science/codex/relay-station-psj-17/,,,yes
+HIP 17694,-72.25,-152.53125,-338.6875,Maia,-81.78125,-149.4375,-343.375,Pleiades Sector GW-W c1-13,-77.375,-156.3125,-352.03125,Pleiades Sector GW-W c1-15,-76.9375,-153.71875,-346.625,Dominic’s Corner,https://canonn.science/codex/dominics-corner/,,,yes
+HIP 17892,-70.75,-157.9375,-352.84375,HR 1185,-64.65625,-148.9375,-330.40625,Pleiades Sector PD-S b4-0,-80.625,-156.6875,-337.4375,HR 1172,-75.1875,-152.40625,-346.59375,Comms Facility 89563,https://canonn.science/codex/comms-facility-89563/,,,yes
+HIP 17892,-70.75,-157.9375,-352.84375,HIP 19072,-54.28125,-121.03125,-323.0625,Pleione,-77.0,-146.78125,-344.125,HIP 19792,-70.15625,-103.21875,-355.84375,Betterton Outpost,https://canonn.science/codex/betterton-outpost/,,,yes
+HIP 19072,-54.28125,-121.03125,-323.0625,Merope,-78.59375,-149.625,-340.53125,Pleiades Sector AB-W b2-4,-137.5625,-118.25,-380.4375,HIP 14909,-84.375,-259.28125,-358.65625,Thargoid Structure,https://canonn.science/codex/hip-14909-distress-call/,,Led to first Thargoid structure found on HIP 14909 2 a. The original distress call no longer exists.,yes
+HIP 19847,-24.84375,-153.46875,-377.46875,HR 1183,-72.875,-145.09375,-336.96875,Pleiades Sector HR-W d1-79,-80.625,-146.65625,-343.25,HIP 19284,-25.40625,-164.875,-365.40625,Site 94,https://canonn.science/codex/site-94/,,,yes
+HIP 21559,-42.09375,-7.40625,-129.5,,,,,,,,,Mentor,-27.65625,-3.5,-85.53125,Vanguard Navigation Beacon,https://canonn.science/codex/eagle-eye-history/,https://elite-dangerous.fandom.com/wiki/Vanguard,"Vanguard was a Flight Operations Carrier Megaship, no sightings since Dec 13, 3304. Believed to be retired by Aegis. Mentioned on Eagle Eye article on Week 3: 22 March 3304.",yes
+HIP 26176,394.4375,-323.53125,-1431.84375,,,,,,,,,Col 69 Sector JI-I c10-4,439.40625,-237.59375,-1208.71875,Commander Hyford storyline (1/4),https://canonn.science/codex/colonia-crash-site/,https://www.youtube.com/watch?v=hisbQoaDox8,"Series of LPs, but story begins at ""Commander Hyford storyline (start)"" in Colonia",yes
+HIP 290,-6.625,-207.8125,38.15625,,,,,,,,,Saffron,72.875,-117.75,55.78125,Omega Grid lore,https://canonn.science/codex/omega-grid/,https://community.elitedangerous.com/galnet/uid/61fbf222bc946221076daa5b,Gives a follow-up inbox msg the next day. Series of beacons with Omega Grid lore with Galnet posts,yes
+HIP 44101,156.6875,22.28125,-33.0625,,,,,,,,,Col 285 Sector WH-O a22-2,143.75,-33.5625,-106.53125,Hesperus beacon TX-B-01,https://canonn.science/codex/proteus/,https://inara.cz/galaxy-logbooks/61035/,"Trail of beacons left behind on the path of the Hesperus, see inara link for an interesting logbook.",yes
+HIP 49538,366.40625,9.5,67.8125,HIP 54181,345.0,1.84375,127.125,Wregoe PV-C c26-16,317.375,31.34375,84.3125,Wregoe JI-B d13-130,336.46875,12.5,93.6875,Site 426,https://canonn.science/codex/site-426/,,,yes
+HIP 98406,-199.03125,-93.03125,241.09375,Swoiwns YM-J b1-6,-128.78125,-112.15625,252.78125,Tseen Foo,-181.78125,-91.25,201.5625,Col 285 Sector EP-C b27-3,-175.03125,-103.03125,232.28125,Unlisted Wreckage Site,https://canonn.science/codex/unlisted-wreckage-site/,,,yes
+Horsehead Sector CB-O b6-0,376.8125,-332.875,-784.84375,,,,,,,,,Flame Sector PI-T b3-0,378.59375,-340.6875,-882.15625,Hesperus beacon TX-B-11,https://canonn.science/codex/proteus/,https://inara.cz/galaxy-logbooks/61035/,"Trail of beacons left behind on the path of the Hesperus, see inara link for an interesting logbook.",yes
+HR 1185,-64.65625,-148.9375,-330.40625,Pleiades Sector HR-W d1-57,-86.40625,-125.15625,-301.84375,,,,,HIP 17746,-43.40625,-180.53125,-337.375,Recon 6 Crash Site (DBX),https://canonn.science/codex/recon-6/,,Eventually leading to a Thargoid Structure in Pleiades Sector OS-U c2-7,yes
+Hyperion,-51.1875,7.5,-16.71875,,,,,,,,,,,,,Memorial for CMDR Adam Taylor,,,Incorrectly shows up as Faction Owned Installation on Nav panel,yes
+Irandan,-86.375,-12.40625,-125.0625,,,,,,,,,Assinda,-70.3125,-118.90625,-112.125,Acropolis Navigation Beacon,https://canonn.science/codex/eagle-eye-history/,https://elite-dangerous.fandom.com/wiki/Acropolis,"Acropolis was a Flight Operations Carrier Megaship, no sightings since Dec 13, 3304. Believed to be retired by Aegis. Mentioned on Eagle Eye article on Week 3: 22 March 3304.",yes
+Jotunheim,66.03125,-110.875,52.6875,,,,,,,,,Sheron,61.40625,182.1875,566,Megaship GCS Sarasvati,https://canonn.science/codex/gcs-sarasvati/,,Partly encoded msg points to system IC 4604 Sector FB-X c1-16 which was renamed to Sheron,yes
+LHS 1047,-3.84375,-15.8125,0.21875,,,,,,,,,LHS 1047,-3.84375,-15.8125,0.21875,Generation Ship Lycaon,https://canonn.science/codex/generation-ship-lycaon/,,,yes
+LHS 1596,-38.5625,14.40625,-38.21875,,,,,,,,,LHS 1596,-38.5625,14.40625,-38.21875,Generation Ship Hyperion,https://canonn.science/codex/generation-ship-hyperion/,,,yes
+LHS 2206,11.40625,25.1875,-18.5,,,,,,,,,Nefertem,38.125,30.875,-21.25,Generation Ship Thetis,https://canonn.science/codex/generation-ship-thetis/,,,yes
+LP 855-10,24.6875,24.46875,22.1875,,,,,,,,,Kitae,-13.5,24.75,-56.46875,Generation Ship Venusian,https://canonn.science/codex/generation-ship-venusian/,,,yes
+LP 926-40,-9.78125,-26.71875,45.1875,,,,,,,,,VESPER-M4,-3.6875,-22.53125,57,Slough Unauthorised Installation,https://canonn.science/codex/slough-unauthorised-installation/,,The start of the trail leading to this listening post was found in the Altair Herald,yes
+Lupus Dark Region AF-Z b1,184.0,121.6875,333.25,Praea Euq FV-Y c12,220.21875,113.65625,282.96875,Praea Euq MR-W c1-20,198.03125,41.59375,332.15625,HR 5625,197.5625,85.40625,322.03125,Trading Vessel MD-003,https://canonn.science/codex/trading-vessel-md-003/,,,yes
+Maia,-81.78125,-149.4375,-343.375,,,,,,,,,Electra,-86.0625,-159.9375,-361.65625,Communication Hub Zeta 12,https://canonn.science/codex/communication-hub-zeta-12/,,Encoded beacon at station further points to Thargoid Structure on HIP 16572 A 4,yes
+Maidjin,33.15625,-85.75,99.71875,,,,,,,,,Zhao Jin,125.25,-43.875,27.53125,Omega Grid lore,https://canonn.science/codex/omega-grid/,,Gives a follow-up inbox msg the next day. Series of beacons with Omega Grid lore with Galnet posts,yes
+Merope,-78.59375,-149.625,-340.53125,Pleiades Sector HR-W d1-74,-87.40625,-150.90625,-344.96875,Pleiades Sector KX-T b3-1,-100.28125,-153.53125,-346.03125,Pleiades Sector IC-U b3-1,-90.1875,-135.15625,-348.3125,Sharpe Works,https://canonn.science/codex/sharpe-works/,,Led to first Thargoid structure found on HIP 14909 2 a. The original distress call no longer exists.,yes
+NGC 1999 Sector HN-S B4-0,455.625,-343.8125,-941.4375,,,,,,,,,NGC 1999 Sector ZU-X b1-0,536.375,-339.3125,-991.59375,Hesperus beacon TX-B-13,https://canonn.science/codex/proteus/,https://inara.cz/galaxy-logbooks/61035/,"Trail of beacons left behind on the path of the Hesperus, see inara link for an interesting logbook.",yes
+NGC 1999 Sector ZU-X b1-0,536.375,-339.3125,-991.59375,,,,,,,,,Trapezium Sector FH-U c3-3,507.25,-336.1875,-1078.125,Hesperus beacon TX-B-14,https://canonn.science/codex/proteus/,https://inara.cz/galaxy-logbooks/61035/,"Trail of beacons left behind on the path of the Hesperus, see inara link for an interesting logbook.",yes
+NGC 2632 Sector IW-W c1-0,258.625,258.1875,-544.75,Wregoe IJ-M b22-0,305.125,260.5,-580.03125,Plio Eurl JI-H b25-0,2445.21875,288.0,-519.875,Wregoe VK-E c12-0,298.125,256.75,-542.21875,Columbus Expedition Camp 14,https://canonn.science/codex/columbus-expedition-camp-14/,,"LPs link to non-existant system Wregoe VK-H b25-0, galaxy map resolves it to Plio Eurl JI-H b25-0",yes
+NLTT 55164,-13.375,-21.65625,6.59375,,,,,,,,,Mu Cassiopeia,-26.125,-24.625,17.34375,Generation Ship Artemis,https://canonn.science/codex/generation-ship-artemis/,,,yes
+Pleiades Sector IH-V c2-5,-78.71875,-138.03125,-321.78125,,,,,,,,,HIP 17125,-95.875,-128.75,-316.5625,Survey Vessel Victoria’s Song,https://canonn.science/codex/survey-vessel-victorias-song/,,(There are two Listening Posts at this body),yes
+Pollux,6.71875,13.71875,-30.125,,,,,,,,,,,,,Memorial for CMDR Tony Pox,,https://forums.frontier.co.uk/threads/clarification-on-pollux-listening-post.421679/,,yes
+Praea Euq AQ-X c1-8,142.59375,227.71875,257.34375,Praea Euq BQ-X C1-11,160.3125,220.0625,306.78125,Praea Euq XJ-Z c0,142.59375,227.71875,257.34375,Praea Euq WO-A d22,139.53125,223.03125,291.5625,Transport Vessel Solomon,https://canonn.science/codex/transport-vessel-solomon/,https://imgur.com/a/f2xYwTz,,yes
+Puppis Sector FB-X b1-4,66.21875,15.21875,-43.46875,,,,,,,,,Hez Ur,149.96875,23.65625,-85.03125,Generation Ship Pleione,https://canonn.science/codex/generation-ship-pleione/,,,yes
+Quiness,-46.46875,37.78125,-24.9375,,,,,,,,,Ross 690,-28.40625,47.8125,-21.96875,Halley Terminal,https://canonn.science/codex/halley-terminal/,https://community.elitedangerous.com/en/galnet/27-JAN-3301,LP points to installation in Karis system but that's an unrelated installation. See Canonn link.,yes
+Ross 446,14.21875,16.90625,-6.53125,,,,,,,,,Istanu,2.0625,55.15625,1.28125,Istanu Unauthorised Installation,https://canonn.science/codex/istanu-unauthorised-installation/,,Start of the trail leading to the LP was found in the Ross 154 Herald,yes
+Saffron,72.875,-117.75,55.78125,,,,,,,,,Maidjin,33.15625,-85.75,99.71875,Omega Grid lore,https://canonn.science/codex/omega-grid/,,Gives a follow-up inbox msg the next day. Series of beacons with Omega Grid lore with Galnet posts,yes
+Shana Bei,-72.1875,-71.375,-59.0625,,,,,,,,,,,,,Memorial for CMDR Tony Voller,,,,yes
+Shinrarta Dezhra,55.71875,17.59375,27.15625,,,,,,,,,,,,,Pilots' Memorial: Shinrarta Dezhra,,https://forums.frontier.co.uk/threads/elite-dangerous-in-game-memorials.566260/,,yes
+Sol,0.0,0.0,0.0,,,,,,,,,,,,,Pilots' Memorial: Sol,,https://forums.frontier.co.uk/threads/elite-dangerous-in-game-memorials.566260/,,yes
+Swoilz TU-R b10-2,241.0625,-118.09375,448.5,Swoilz XR-W c4-31,311.09375,-133.15625,416.90625,Swoilz TZ-R b10-4,285.0,-93.90625,448.71875,HIP 83003,280.875,-119,434.875,Trading Vessel MD-004,https://canonn.science/codex/trading-vessel-md-004/,,,yes
+Synuefai JI-R d5-12,-926.125,-464.34375,-572.71875,Synuefai ML-U b20-0,-824.03125,-537.5,-606.4375,Synuefai SS-Y b18-0,-902.375,-463.3125,-664.6875,Synuefai FV-U b20-0,-879.5,-499.875,-619.1875,Extraction Site V-81,https://canonn.science/codex/extraction-site-v-81/,,,yes
+Synuefai VJ-C b19-1,-69.75,-69.28125,-658.6875,Synuefe CV-P c8-11,-30.65625,-67.625,-696.71875,Synuefe TI-A b20-1,-4.375,-99.3125,-642.15625,Synuefe QA-U d4-27,-37.625,-80.09375,-664.71875,Pirate Cache,https://canonn.science/codex/pirate-cache/,,,yes
+Synuefe BA-X B34-2,198.65625,-214.125,-309.8125,,,,,,,,,Synuefe JJ-O d7-55,191.5625,-259.1875,-391.71875,Hesperus beacon TX-B-05,https://canonn.science/codex/proteus/,https://inara.cz/galaxy-logbooks/61035/,"Trail of beacons left behind on the path of the Hesperus, see inara link for an interesting logbook.",yes
+Synuefe FM-S B37-4,208.71875,-138.84375,-255.4375,,,,,,,,,Synuefe BA-X B34-2,198.65625,-214.125,-309.8125,Hesperus beacon TX-B-04,https://canonn.science/codex/proteus/,https://inara.cz/galaxy-logbooks/61035/,"Trail of beacons left behind on the path of the Hesperus, see inara link for an interesting logbook.",yes
+Synuefe GB-O c9-8,-48.78125,-72.21875,-656.0625,,,,,,,,,HIP 22460,-41.3125,-58.96875,-354.78125,Commander Hyford storyline (4/4),https://canonn.science/codex/colonia-crash-site/,https://www.youtube.com/watch?v=hisbQoaDox8,"Scanning this LP will give the HIP 22460 permit, where you'll find the Overlook megaship & Fort Asch",yes
+Synuefe HY-P D6-31,215.96875,-306.125,-468.40625,,,,,,,,,Synuefe MQ-H C12-4,268.21875,-328.25,-544.3125,Hesperus beacon TX-B-07,https://canonn.science/codex/proteus/,https://inara.cz/galaxy-logbooks/61035/,"Trail of beacons left behind on the path of the Hesperus, see inara link for an interesting logbook.",yes
+Synuefe JJ-O d7-55,191.5625,-259.1875,-391.71875,,,,,,,,,Synuefe HY-P D6-31,215.96875,-306.125,-468.40625,Hesperus beacon TX-B-06,https://canonn.science/codex/proteus/,https://inara.cz/galaxy-logbooks/61035/,"Trail of beacons left behind on the path of the Hesperus, see inara link for an interesting logbook.",yes
+Synuefe KN-A a82-0,377.6875,-44.5,-194.625,Wregoe DZ-D b39-6,283.90625,-5.625,-208.03125,Wregoe PD-K d8-40,342.21875,-22.5,-268.25,Synuefe KN-J a77-3,342.59375,-29.1875,-236.78125,Small surface base,https://canonn.science/codex/kidnapped/,https://forums.frontier.co.uk/threads/just-found-probably-undescovered-yet-abandoned-settlement.580939/,,yes
+Synuefe MQ-H C12-4,268.21875,-328.25,-544.3125,,,,,,,,,Synuefe RJ-U B21-0,330.46875,-357.65625,-603.4375,Hesperus beacon TX-B-08,https://canonn.science/codex/proteus/,https://inara.cz/galaxy-logbooks/61035/,"Trail of beacons left behind on the path of the Hesperus, see inara link for an interesting logbook.",yes
+Synuefe RJ-U B21-0,330.46875,-357.65625,-603.4375,,,,,,,,,Witch Head Sector OT-Q b5-0,337.625,-359.71875,-701.125,Hesperus beacon TX-B-09,https://canonn.science/codex/proteus/,https://inara.cz/galaxy-logbooks/61035/,"Trail of beacons left behind on the path of the Hesperus, see inara link for an interesting logbook.",yes
+Synuefe XE-Y c17-7,218.78125,-102.4375,-279.34375,,,,,,,,,,,,,Adamastor Listening Post,https://canonn.science/codex/adamastor-listening-post/,https://forums.frontier.co.uk/threads/cryptic-elite-instagram-twitter-posts.557207/post-8780281,Partially unsolved - Encoded message has not been fully decrypted. Believed to point to Site 23B.,partially
+Taurus Dark Region UY-R b4-0,-3.59375,-120.4375,-444.5625,Perseus Dark Region LN-K b8-0,-363.78125,-380.78125,-974.53125,California Sector IH-V c2-1,-352.03125,-222.65625,-885.21875,Perseus Dark Region KC-V c2-2,-368.03125,-321.6875,-1047.625,Hesperus,https://canonn.science/codex/hesperus/,,"Encrypted message, points to NGC 1333 and California Sectors",yes
+Teorge,79.4375,60.65625,74.3125,,,,,,,,,,,,,Ragazza Logs (7 LPs),https://canonn.science/codex/teorge-listening-posts/,,"Recordings seems to be made by Rebecca, who also made the logs on megaship The Zurara.",yes
+Thoth,1.9375,41.46875,-24.8125,,,,,,,,,,,,,Transport Lakon Baker Gamma Sierra Heavy,https://canonn.science/codex/transport-lakon-baker-gamma-sierra-heavy/,,Distress Beacon points to crashed ship on the moon. An easter egg from a livestream with developer CMDR Dav.,yes
+Trapezium Sector FH-U c3-3,507.25,-336.1875,-1078.125,,,,,,,,,Col 69 Sector VK-E c12-10,440.84375,-309.40625,-1136.9375,Hesperus beacon TX-B-15,https://canonn.science/codex/proteus/,https://inara.cz/galaxy-logbooks/61035/,"Trail of beacons left behind on the path of the Hesperus, see inara link for an interesting logbook.",yes
+Upaniklis,-151.21875,-25.0,77.71875,,,,,,,,,,,,,Megaship The Golconda,https://canonn.science/codex/the-golconda/,https://community.elitedangerous.com/galnet/uid/5dc583285c6f2e59a851f5d0,Broken up message leads to The Golconda in the same system at B 7,yes
+Varati,-178.65625,77.125,-87.125,,,,,,,,,Synuefe QM-I b43-10,590.96875,-81.03125,-135.96875,Jonas Inc Relay Station C-64 & The Bifrost,,https://imgur.com/gallery/fLetr,"Canonn rescue mission. Points to Jonas Inc Relay Station C-64 where CMDR Lady Outspan directed you to a lost expedition on Col 173 Sector LJ-F C12-0 (A 4 c) named The Bifrost. (Also megaship The Cete in same system, and Guardian sites at B 6 and B 7)",yes
+Witch Head Sector OT-Q b5-0,337.625,-359.71875,-701.125,,,,,,,,,Horsehead Sector CB-O b6-0,376.8125,-332.875,-784.84375,Hesperus beacon TX-B-10,https://canonn.science/codex/proteus/,https://inara.cz/galaxy-logbooks/61035/,"Trail of beacons left behind on the path of the Hesperus, see inara link for an interesting logbook.",yes
+Wolf 1301,-15.4375,-34.6875,-79.40625,,,,,,,,,,,,,Memorial Family is More than Blood,,https://eddb.io/attraction/73890,Memorial for CMDR Hirobadger,yes
+Wredguia EB-F d11-37,-495.0625,93.53125,-87.6875,Wredguia EB-M c21-11,-457.59375,100.125,-129.28125,Wredguia KC-K c22-1,-437.34375,93.9375,-100.28125,Wredguia JC-K c22-8,-469.4375,93.625,-104.21875,Colony SNB-86,https://canonn.science/codex/colony-snb-86/,,,yes
+Wredguia DQ-R b20-0,-557.34375,451.125,-617.625,Wredguia QO-K b24-0,-612.9375,445.125,-543.6875,Wredguia RI-S d4-10,-618.84375,484.875,-636.125,Wredguia RI-S d4-4,-595.125,460.59375,-598.53125,Lazarus Outpost,https://canonn.science/codex/lost-explorer/,,,yes
+Wredguia ML-P d5-21,39.375,-513.59375,558.59237,Wredguia PR-N d6-28,47.21875,-454.34375,538.44079,Wredguia UX-L d7-53,-2.15625,-421.46875,471.52217,HIP 20556,-229.40625,19.6875,-447.59375,Abandoned SRV,,https://imgur.com/a/DJTGlwp,,yes
+Wregoe CU-B b15-0,60.9375,400.875,-728.15625,Wregoe NW-X b16-0,40.5625,356.3125,-696.40625,Wregoe YY-B b15-0,18.78125,417.3125,-740.28125,Wregoe HV-Z b15-0,38.15625,388.5625,-719.84375,Herpin Research Base,https://canonn.science/codex/herpin-research-base/,,,yes
+Wregoe DK-R b4-1,171.65625,7.5625,-951.1875,,,,,,,,,Synuefe Gb-O c9-8,-48.78125,-72.21875,-656.0625,Commander Hyford storyline (3/4),https://canonn.science/codex/colonia-crash-site/,https://www.youtube.com/watch?v=hisbQoaDox8,"Series of LPs, but story begins at ""Commander Hyford storyline (start)"" in Colonia",yes
+Wregoe TA-E d12-6,197.375,381.96875,48.59375,Wregoe IB-Z c28-1,196.1875,358.25,177.46875,Wregoe YB-C d13-36,152.03125,304.15625,106.53125,HIP 62233,183.875,353.65625,111.40625,Trading Vessel MD-001,https://canonn.science/codex/trading-vessel-md-001/,,,yes
+Zhao Jin,125.25,-43.875,27.53125,,,,,,,,,,,,,Omega Grid lore,https://canonn.science/codex/omega-grid/,https://community.elitedangerous.com/galnet/uid/6203a754f628791f254db8d8,Gives a follow-up inbox msg the next day. Series of beacons with Omega Grid lore with Galnet posts,yes
\ No newline at end of file
diff --git a/data/csvCache/Thargoid Surface Site Survey (Responses) - Form responses 1.csv b/data/csvCache/Thargoid Surface Site Survey (Responses) - Form responses 1.csv
new file mode 100644
index 0000000..8c80e57
--- /dev/null
+++ b/data/csvCache/Thargoid Surface Site Survey (Responses) - Form responses 1.csv
@@ -0,0 +1,1791 @@
+Timestamp,Commander,System,Planet,Aerial Screenshot,Leviathans,Doors,Internal Doors,Device,Link System 1,Link System 2,Link System 3,Notes,Visibility,Blockages,Leviathan Count,,
+17/12/2018 22:22:58,JohnTAss,HIP 17862,11 E,https://i.imgur.com/gYl7PRC.png,Small Reposing Leviathans,2,No,No,,,,,1,,1,,
+17/12/2018 22:25:07,JohnTAss,HIP 17694,B 7 F,https://i.imgur.com/8PIKHUU.png,Small Reposing Leviathans,2,No,No,,,,,5,,2,,
+17/12/2018 22:49:02,JohnTAss,Pleiades Sector OD-S b4-1,2 A,https://i.imgur.com/2bBcw7N.png,Large upright leviathans,3,No,Yes,,,,System link from thargoid machine overwritten by Eagle Eye (2 messages),1,,4,,
+17/12/2018 23:21:06,JohnTAss,HIP 16704,5 A,https://i.imgur.com/OiC4oO2.jpg,Small Reposing Leviathans,3,No,Yes,,,,Eagle Eye overwrote messages,5,,4,,
+17/12/2018 23:47:47,JohnTAss,Mel 22 Sector JC-V d2-14,A 4 A,https://i.imgur.com/ETPSWvO.png,Large upright leviathans,4,Yes,Yes,,,,Eagle Eye wrote over the original messages,1,,6,,
+18/12/2018 00:09:26,JohnTAss,Mel 22 Sector JC-V d2-15,ABC 1 A,https://i.imgur.com/BhlGOqa.png,Large upright leviathans,4,Yes,Yes,MEL 22 SECTOR JC-V D2-14,MEL 22 SECTOR JC-V D2-41,MEL 22 SECTOR IO-G B11-1,In shadow of mountain,1,,6,,
+18/12/2018 00:31:14,JohnTAss,Mel 22 Sector OY-R c4-5,2 A,https://i.imgur.com/waAh26z.png,Large upright leviathans,4,Yes,Yes,MEL 22 SECTOR IO-G B11-1,MEL 22 SECTOR JC-V D2-15,MEL 22 SECTOR JC-V D2-14,,1,,5,,
+18/12/2018 00:52:53,JohnTAss,Mel 22 Sector IO-G b11-1,1 A,https://i.imgur.com/75GMkmL.png,Large upright leviathans,4,Yes,Yes,,,,Eagle Eye overwrote signals here too,1,,4,,
+18/12/2018 01:23:10,JohnTAss,Mel 22 Sector JC-V d2-31,A 16 B,https://i.imgur.com/zgwCYSL.png,Large upright leviathans,4,Yes,Yes,,,,EE overwrote; in shadow of crater wall.,2,,6,,
+18/12/2018 01:49:48,JohnTAss,Mel 22 Sector HH-V d2-37,AB 1 A,https://i.imgur.com/Ux9f5H0.png,Small Reposing Leviathans,4,Yes,Yes,,,,EE overwrote messages.,1,,3,,
+18/12/2018 02:11:12,JohnTAss,Mel 22 Sector HH-V d2-53,1 B,https://i.imgur.com/smJKGoQ.png,Small Reposing Leviathans,3,No,Yes,TAURUS DARK REGION MY-R B4-1,MEL 22 SECTOR JX-T C3-9,TAURUS DARK REGION CL-Y D53,,4,,4,,
+18/12/2018 16:23:46,Rufus Nightjar,HIP 17862,11 E,https://i.imgur.com/ulDQD34.jpg,,0,,No,,,,,3,,1,,
+18/12/2018 17:34:47,Rufus Nightjar,HIP 17694,B 7 F,,,2,,No,,,,,3,,2,,
+18/12/2018 20:22:15,JohnTAss,Mel 22 Sector FH-U c3-9,A 1 A,https://i.imgur.com/ljWb7ry.png,Large upright leviathans,4,Yes,Yes,,,,EE overwrote; alsdo inside doors didnt load until I got the Link. probably a bug.,2,,6,,
+18/12/2018 20:40:47,JohnTAss,Taurus Dark Region MY-R b4-1,1 B,https://i.imgur.com/lvSgMht.png,Large upright leviathans,4,Yes,Yes,MEL 22 SECTOR HH-V D2-53,MEL 22 SECTOR FH-U C3-9,TAURUS DARK REGION CL-Y D53,,3,,5,,
+18/12/2018 20:58:14,JohnTAss,Taurus Dark Region CL-Y d53,A 4,https://i.imgur.com/zGAZTtq.png,Small Reposing Leviathans,4,Yes,Yes,,,,EE overwrote messages,3,,3,,
+18/12/2018 21:34:48,JohnTAss,Mel 22 Sector DB-X d1-46,A 8 A,https://i.imgur.com/Ni3Zbjc.jpg,Small Reposing Leviathans,4,Yes,Yes,,,,EE overwrote,5,,4,,
+18/12/2018 21:50:47,JohnTAss,Mel 22 Sector JX-T c3-9,6 A,https://i.imgur.com/O843nKf.png,Small Reposing Leviathans,4,Yes,Yes,,,,EE overwrote,1,,5,,
+18/12/2018 22:26:57,JohnTAss,HIP 15889,11 B,https://i.imgur.com/qrdOp5i.png,Small Reposing Leviathans,4,Yes,Yes,HIP 16704,MEL 22 SECTOR JX-T C3-9,MEL 22 SECTOR HH-V D2-37,,1,,4,,
+18/12/2018 22:45:55,JohnTAss,HIP 16795,A 10 F,https://i.imgur.com/YxFw2fV.png,Small Reposing Leviathans,4,Yes,Yes,MEL 22 SECTOR DB-X D1-46,HIP 15889,TAURUS DARK REGION CL-Y D53,,1,,3,,
+18/12/2018 23:01:40,JohnTAss,Taurus Dark Region FW-W c1-3,1 A,https://i.imgur.com/gOwnw6p.png,Small Reposing Leviathans,4,Yes,Yes,MEL 22 SECTOR IS-S C4-6,TAURUS DARK REGION MY-R B4-1,TAURUS DARK REGION DL-Y D54,,2,,5,,
+18/12/2018 23:19:55,JohnTAss,Taurus Dark Region BQ-Y c12,2 A,https://i.imgur.com/2AgUHjW.png,Large upright leviathans,4,Yes,Yes,HIP 21373,HIP 20989,TAURUS DARK REGION DL-Y D54,,1,,5,,
+19/12/2018 16:22:02,JohnTAss,Hind Sector GR-W d1-13,8 H,https://i.imgur.com/kvoZUhP.png,No Leviathans,0,No,No,,,,,1,,2,,
+19/12/2018 16:42:34,JohnTAss,HIP 19665,8 B,https://i.imgur.com/SXTlmga.png,Small Reposing Leviathans,4,Yes,Yes,PLEIADES SECTOR DQ-Y C4,HIP 20644,TAURUS DARK REGION QN-T B3-0,,1,,3,,
+19/12/2018 16:59:22,JohnTAss,Pleiades Sector DQ-Y c4,6 A,https://i.imgur.com/opLVA3J.png,Small Reposing Leviathans,4,Yes,Yes,,,,EE overwrote,2,,5,,
+19/12/2018 17:17:00,JohnTAss,Pleiades Sector GG-Y d33,3 A,https://i.imgur.com/OtoDITY.png,Small Reposing Leviathans,4,Yes,Yes,,,,EE overwrote,1,,6,,
+19/12/2018 17:32:35,JohnTAss,Struve's Lost Sector OI-T c3-3,2 B,https://i.imgur.com/BSCRrzc.png,Large upright leviathans,4,Yes,Yes,PLEIADES SECTOR GG-Y D33,HIP 18117,PLEIADES SECTOR DQ-Y C4,,1,,4,,
+19/12/2018 17:52:49,JohnTAss,HIP 16985,A 4 E,https://i.imgur.com/zGOeISj.png,Large upright leviathans,4,Yes,Yes,MEL 22 SECTOR UE-G B11-0,MEL 22 SECTOR BV-P C5-2,MEL 22 SECTOR KC-V D2-28,,1,,6,,
+19/12/2018 18:10:25,JohnTAss,Mel 22 Sector MX-U d2-14,AB 1 A,https://i.imgur.com/nBz6AAG.png,Large upright leviathans,4,Yes,Yes,,,,EE overwrote,2,,3,,
+19/12/2018 18:27:54,JohnTAss,Mel 22 Sector TT-R c4-2,3 A,https://i.imgur.com/xUT6rTM.png,Large upright leviathans,4,Yes,Yes,,,,EE overwrote,2,,6,,
+19/12/2018 20:37:14,Rufus Nightjar,Pleiades Sector OD-S b4-1,2 A,https://i.imgur.com/MEGKPdJ.jpg,,3,No,Yes,,,,,2,,4,,
+20/12/2018 00:08:40,JohnTAss,Mel 22 Sector KC-V d2-28,1 C,https://i.imgur.com/y8rHti4.png,Large upright leviathans,4,Yes,Yes,,,,EE overwrote,4,,5,,
+20/12/2018 00:24:24,JohnTAss,Mel 22 Sector NX-U d2-31,3 A,https://i.imgur.com/RtodgKq.png,Large upright leviathans,4,Yes,Yes,,,,EE overwrote,1,,5,,
+20/12/2018 00:41:43,JohnTAss,Mel 22 Sector NX-U d2-27,5 A,https://i.imgur.com/29E5sGa.png,Small Reposing Leviathans,4,No,Yes,MEL 22 SECTOR YU-F B11-1,MEL 22 SECTOR NX-U D2-31,MEL 22 SECTOR ZU-P C5-1,,1,,4,,
+20/12/2018 00:53:23,Johnson sky,HIP 22711,7 A,https://inara.cz/cmdr-gallery/55415/,Large upright leviathans,4,yes,Yes,,,,,1,,3,,
+20/12/2018 00:57:32,JohnTAss,Mel 22 Sector ZU-P c5-1,4 A,https://i.imgur.com/pGRERJA.png,Large upright leviathans,4,Yes,Yes,,,,EE overwrote,1,,4,,
+20/12/2018 01:14:27,JohnTAss,HIP 14909,2 A,https://i.imgur.com/CtbmV9K.png,Small Reposing Leviathans,3,No,Yes,,,,EE overwrote,4,,4,,
+20/12/2018 01:30:33,TK-421 Bowdog,HIP 17862,11 E,,Small Reposing Leviathans,4,Unsure,No,,,,,1,,1,,
+20/12/2018 03:49:52,JohnTAss,Mel 22 Sector CB-O c6-4,3 A,https://i.imgur.com/5Yy0kJc.png,Large upright leviathans,3,No,Yes,PLEIADES SECTOR OS-U C2-6,HIP 14909,MEL 22 SECTOR SK-E B12-1,,2,,3,,
+20/12/2018 04:06:07,JohnTAss,Pleiades Sector OS-U c2-6,A 5 B,https://i.imgur.com/wFBPmwa.png,Large upright leviathans,4,Yes,Yes,PLEIADES SECTOR KM-W D1-40,HIP 14909,MEL 22 SECTOR CB-O C6-4,,1,,4,,
+20/12/2018 04:21:08,JohnTAss,Pleiades Sector OS-U c2-7,4 A,https://i.imgur.com/h5yWbhv.png,No Leviathans,0,No,No,,,,,1,,0,,
+20/12/2018 04:45:02,JohnTAss,Pleiades Sector JM-W d1-52,2 A,https://i.imgur.com/7PkRSYQ.png,Small Reposing Leviathans,3,No,Yes,PLEIADES SECTOR OD-S B4-1,PLEIADES SECTOR OS-U C2-7,PLEIADES SECTOR OS-U C2-6,,1,,4,,
+20/12/2018 04:53:02,,HIP 17862,11 E,,Large upright leviathans,,,No,,,,,0,,1,,
+20/12/2018 11:38:13,,HIP 17694,B 7 F,,Small Reposing Leviathans,4,,,,,,Multiple scavengers were present,0,,2,,
+21/12/2018 09:02:23,JohnTAss,Pleiades Sector LX-U c2-2,AB 4 A,https://i.imgur.com/hr6SjcR.png,Small Reposing Leviathans,3,No,Yes,,,,EE overwrote,2,,4,,
+21/12/2018 09:23:09,JohnTAss,Pleiades Sector JC-V c2-9,1 A,https://i.imgur.com/HrdVgCN.png,Small Reposing Leviathans,3,No,Yes,HIP 15746,PLEIADES SECTOR HH-V C2-6,PLEIADES SECTOR HH-V C2-13,Got Medusa visitor on exit,4,,4,,
+21/12/2018 09:41:03,JohnTAss,Pleiades Sector HH-V c2-13,A 1 C,https://i.imgur.com/8UZXFjE.png,Small Reposing Leviathans,3,No,Yes,,,,EE overwrote,4,,4,,
+21/12/2018 10:38:32,JohnTAss,Pleiades Sector KT-Q b5-3,5 A,https://i.imgur.com/840ldeX.png,Small Reposing Leviathans,4,Yes,Yes,HIP 19026,PLEIADES SECTOR EC-U B3-0,HYADES SECTOR XO-A C15,,1,,5,,
+21/12/2018 10:57:14,JohnTAss,Pleiades Sector EC-U b3-0,4 A,https://i.imgur.com/ZAsdwUY.png,Small Reposing Leviathans,3,Yes,Yes,MEL 22 SECTOR OO-Q C5-6,PLEIADES SECTOR KT-Q B5-3,HYADES SECTOR XO-A C15,"There is a door in the outer circle, not into the center chamber",1,,4,,
+21/12/2018 11:23:44,JohnTAss,HIP 15746,AB 1 B,https://i.imgur.com/Iov3KLr.png,Large upright leviathans,4,Yes,Yes,PLEIADES SECTOR LN-T C3-11,HIP 16704,PLEIADES SECTOR EC-U B3-0,,1,,6,,
+21/12/2018 11:40:27,JohnTAss,Mel 22 Sector SK-E b12-1,2,https://i.imgur.com/r8uCUIz.jpg,Large upright leviathans,4,Yes,Yes,MEL 22 SECTOR CB-O C6-4,MEL 22 SECTOR JC-V D2-14,MEL 22 SECTOR JC-V D2-15,,3,,3,,
+21/12/2018 11:57:21,JohnTAss,Mel 22 Sector JC-V d2-41,A 1 A,https://i.imgur.com/IcRbDPQ.png,Large upright leviathans,4,Yes,Yes,,,,EE overwrote,,,4,,
+21/12/2018 12:13:17,JohnTAss,Mel 22 Sector JC-V d2-19,4 B,https://i.imgur.com/DRKw3Ig.jpg,Large upright leviathans,4,Yes,Yes,,,,EE overwrote,3,,3,,
+21/12/2018 12:27:08,JohnTAss,Mel 22 Sector AX-A b14-1,1 B,https://i.imgur.com/wZBswPp.png,No Leviathans,0,No,No,,,,,,,0,,
+21/12/2018 13:51:27,JohnTAss,Aries Dark Region HR-W c1-1,8 C,https://i.imgur.com/MHlnw7v.png,Small Reposing Leviathans,3,No,Yes,,,,EE overwrote,,,4,,
+21/12/2018 14:07:59,JohnTAss,Mel 22 Sector WK-O c6-1,5 C,https://i.imgur.com/Q0vGsqm.png,Large upright leviathans,4,Yes,Yes,,,,EE overwrote,,,5,,
+21/12/2018 14:25:11,JohntAss,Synuefai YQ-X c17-8,3 A,https://i.imgur.com/kkAwiTJ.png,Large upright leviathans,4,Yes,Yes,SYNUEFAI YQ-X C17-7,SYNUEFAI UA-Y C17-4,ARIES DARK REGION DB-X C1-2,,,,4,,
+21/12/2018 15:09:08,JohnTAss,Synuefai YQ-X c17-7,1 A,https://i.imgur.com/nEly3z9.png,Large upright leviathans,4,Yes,Yes,SYNUEFAI WV-X C17-12,SYNUEFAI YQ-X C17-8,ARIES DARK REGION HR-W D1-44,,,,3,,
+21/12/2018 22:16:40,JohnTAss,Synuefai WV-X c17-12,2 B,https://i.imgur.com/lk1bmVA.png,Small Reposing Leviathans,3,No,Yes,SYNUEFAI YQ-X C17-7,PLEIADES SECTOR KT-Q B5-3,SYNUEFAI YQ-X C17-8,,,,4,,
+21/12/2018 22:39:36,JohnTAss,Mel 22 Sector LN-T d3-60,5 A,https://i.imgur.com/RkREgrj.png,Small Reposing Leviathans,3,No,Yes,ARIES DARK REGION DB-X C1-2,SYNUEFAI YQ-X C17-7,SYNUEFAI WV-X C17-12,,,,4,,
+21/12/2018 23:03:53,JohnTAss,Aries Dark Region DB-X c1-2,B 1,https://i.imgur.com/HvP9M3A.png,Small Reposing Leviathans,3,No,Yes,ARIES DARK REGION DB-X C1-7,MEL 22 SECTOR LN-T D3-60,MEL 22 SECTOR WK-O C6-1,Basi visit on site exit,,,4,,
+21/12/2018 23:21:05,JohnTAss,Aries Dark Region DB-X c1-7,A 9 A,https://i.imgur.com/ngfUpLE.png,Small Reposing Leviathans,3,No,Yes,ARIES DARK REGION DB-X C1-2,ARIES DARK REGION AQ-Y D14,MEL 22 SECTOR LN-T D3-60,"1 door in outer ring, no doors to inner chamber",,,4,,
+21/12/2018 23:30:01,LCU No Fool Like One,HIP 17862,11 E,https://i.imgur.com/DAigxzy.jpg,Small Reposing Leviathans,2,No,No,,,,,,,1,,
+21/12/2018 23:45:33,JohnTAss,Synuefai UA-Y c17-4,7 A,https://i.imgur.com/ox5Q0fh.png,Small Reposing Leviathans,4,Yes,Yes,SYNUEFAI WV-X C17-12,MEL 22 SECTOR LN-T D3-64,ARIES DARK REGION HR-W D1-54,,,,3,,
+22/12/2018 00:15:20,JohnTAss,Mel 22 Sector LN-T d3-64,ABC 1 C,https://i.imgur.com/u7ttqmO.png,Large upright leviathans,4,Yes,Yes,MEL 22 SECTOR OO-Q C5-6,SYNUEFAI UA-Y C17-4,MEL 22 SECTOR OO-Q C5-10,,,,5,,
+22/12/2018 01:38:02,LCU No Fool Like One,HIP 17694,B 7 F,https://i.imgur.com/9wlvjlj.png,Small Reposing Leviathans,2,,No,,,,,,,2,,
+22/12/2018 08:18:07,JohnTAss,Mel 22 Sector OO-Q c5-6,1 A,https://i.imgur.com/72HMpoR.png,Large upright leviathans,4,Yes,Yes,,,,EE overwrote,,,5,,
+22/12/2018 08:36:09,JohnTAss,Mel 22 Sector OO-Q c5-10,7 B,https://i.imgur.com/RMmCBHQ.png,Large upright leviathans,4,Yes,Yes,MEL 22 SECTOR FM-V D2-67,MEL 22 SECTOR LN-T D3-64,MEL 22 SECTOR OO-Q C5-6,,,,5,,
+22/12/2018 08:54:44,JohnTAss,Mel 22 Sector FM-V d2-67,2 A,https://i.imgur.com/62E5dny.png,Large upright leviathans,4,Yes,Yes,,,,EE overwrote,,,4,,
+22/12/2018 09:25:38,JohnTAss,HIP 14969,CD 2 A,https://i.imgur.com/Lqvs8iq.png,Small Reposing Leviathans,3,No,Yes,SYNUEFAI RK-Y C17-3,COL 285 SECTOR BQ-X C1-10,SYNUEFAI UZ-M D8-63,One door on inside ring,,,4,,
+22/12/2018 09:43:30,JohnTAss,Mel 22 Sector NT-Q c5-11,8 A,https://i.imgur.com/Xlr92I2.png,Small Reposing Leviathans,4,Yes,Yes,MEL 22 SECTOR ZM-H B11-3,MEL 22 SECTOR FM-V D2-38,HIP 14756,,,,4,,
+22/12/2018 17:20:01,JohnTAss,Mel 22 Sector ZM-H b11-3,B 3,https://i.imgur.com/VgoT6Jc.png,Large upright leviathans,4,Yes,Yes,MEL 22 SECTOR NT-Q C5-11,MEL 22 SECTOR FM-V D2-38,MEL 22 SECTOR FM-V D2-67,,,,5,,
+22/12/2018 17:38:10,JohnTAss,Mel 22 Sector FM-V d2-38,A 3 A,https://i.imgur.com/zng5Ej5.png,Large upright leviathans,4,Yes,Yes,,,,EE overwrote; Medusa visit on exit,,,3,,
+22/12/2018 18:00:16,JohnTAss,Pleiades Sector BG-X c1-14,5 D,https://i.imgur.com/AL6lGGO.png,Small Reposing Leviathans,3,No,Yes,PLEIADES SECTOR BG-X C1-10,MEL 22 SECTOR FM-V D2-38,PLEIADES SECTOR UZ-X B1-2,,,,4,,
+22/12/2018 18:17:33,JohnTAss,Pleiades Sector UZ-X b1-2,A 1 C,https://i.imgur.com/8Oo3VXy.png,No Leviathans,0,No,No,,,,,,,0,,
+23/12/2018 08:57:54,JohnTAss,Pleiades Sector BG-X c1-10,14 A,https://i.imgur.com/8nsCX2K.png,Large upright leviathans,4,Yes,Yes,,,,EE overwrote,,,3,,
+23/12/2018 09:23:51,JohnTAss,Pleiades Sector IX-S b4-1,10 A,https://i.imgur.com/nnhzUH0.png,Large upright leviathans,3,No,Yes,HIP 23022,PLEIADES SECTOR DG-X C1-14,HIP 22382,,,,4,,
+23/12/2018 09:39:32,JohnTAss,Pleiades Sector DG-X c1-11,A 3A,https://i.imgur.com/SKjAqzV.png,Small Reposing Leviathans,0,No,No,,,,,,,1,,
+23/12/2018 09:55:55,JohnTAss,Pleiades Sector DG-X c1-14,AB 1 A,https://i.imgur.com/tlVzoJ4.png,Small Reposing Leviathans,3,No,Yes,HIP 22334,HIP 24934,HIP 21348,,,,4,,
+23/12/2018 10:13:57,JohnTAss,HIP 24007,1 B,https://i.imgur.com/p017vVg.png,Small Reposing Leviathans,3,No,Yes,HIP 22382,SYNUEFE LD-A C17-3,SYNUEFE LD-A C17-1,,,,4,,
+23/12/2018 10:50:43,JohnTAss,Synuefe LD-A c17-1,B 1 C,https://i.imgur.com/7t3MpLb.png,Small Reposing Leviathans,3,No,Yes,SYNUEFE LD-A C17-3,COL 285 SECTOR IL-X C1-4,HIP 25274,,,,4,,
+23/12/2018 11:10:52,JohnTAss,Synuefe LD-A c17-3,AB 2 F,https://i.imgur.com/9fux0d6.png,Small Reposing Leviathans,3,No,Yes,PLEIADES SECTOR IM-V C2-16,HIP 22118,SYNUEFE LD-A C17-1,,,,4,,
+23/12/2018 11:28:02,JohnTAss,HIP 22382,5 E,https://i.imgur.com/TNvsB6K.png,Small Reposing Leviathans,3,No,Yes,COL 285 SECTOR CV-Y D71,COL 285 SECTOR CV-Y D57,HIP 23022,,,,4,,
+23/12/2018 11:49:41,JohnTAss,HIP 23022,B 2 A,https://i.imgur.com/pCKHPQF.png,Small Reposing Leviathans,3,No,Yes,SYNUEFE LD-A C17-3,SYNUEFE LD-A C17-1,COL 285 SECTOR YO-Z C19,,,,4,,
+23/12/2018 12:03:22,JohnTAss,Col 285 Sector CV-Y d71,5 D,https://i.imgur.com/3N79SkY.png,Small Reposing Leviathans,0,No,No,,,,,,,2,,
+27/12/2018 12:21:30,Leadr0fthepack,HIP 17862,11 E,https://screenshotscontent-d5001.xboxlive.com/xuid-2533274938631819-private/d14f04d9-b7ff-46f7-bda4-3ad6409ae96a.PNG?sv=2015-12-11&sr=b&si=DefaultAccess&sig=nPPUeur5t4q9sVbQBAb24j4XRLFLINfBdmgj%2FS5TASQ%3D,Small Reposing Leviathans,1,0,No,N/A,N/A,N/A,"I met a traveller from an antique land,
+Who said—“Two vast and trunkless legs of stone
+Stand in the desert. . . . Near them, on the sand,
+Half sunk a shattered visage lies, whose frown,
+And wrinkled lip, and sneer of cold command,
+Tell that its sculptor well those passions read
+Which yet survive, stamped on these lifeless things,
+The hand that mocked them, and the heart that fed;
+And on the pedestal, these words appear:
+My name is Ozymandias, King of Kings;
+Look on my Works, ye Mighty, and despair!
+Nothing beside remains. Round the decay
+Of that colossal Wreck, boundless and bare
+The lone and level sands stretch far away.",,,1,,
+27/12/2018 12:52:37,Leadr0fthepack,HIP 17694,B 7 F,,Large upright leviathans,4,N/A,No,N/A,N/A,N/A,this planet is completely devoid of any interesting geology and biology apart from the thargoid site,,,2,,
+29/12/2018 00:36:10,,Synuefe HZ-M d8-69,B 7 f,https://i.imgur.com/UDl7LHA.png,Large upright leviathans,3,,Yes,,,Only 2 Links,One door and corridor blocked,,,4,,
+30/12/2018 00:58:23,JohnTAss,Col 285 Sector ZZ-Y d22,2 A,https://i.imgur.com/8bC2isn.png,Small Reposing Leviathans,0,No,No,,,,,,,2,,
+30/12/2018 01:16:44,JohnTAss,Col 285 Sector YO-Z c19,B 2 A,https://i.imgur.com/3ZK4S18.png,Small Reposing Leviathans,0,No,No,,,,,,,2,,
+30/12/2018 01:35:34,JohnTAss,HIP 24934,A 3 A,https://i.imgur.com/8lFpSZg.png,Small Reposing Leviathans,,No,No,,,,,,,1,,
+30/12/2018 01:59:07,JohnTAss,Wregoe RA-W c15-18,A 1 A,https://i.imgur.com/05R8b2d.png,Small Reposing Leviathans,3,No,Yes,,,,EE overwrote messages,,,4,,
+30/12/2018 02:19:04,JohnTAss,HIP 23269,A 1 C,https://i.imgur.com/kuQfxsh.png,Small Reposing Leviathans,3,No,Yes,TAURUS DARK REGION DB-X D1-26,WREDGUIA VX-L D7-80,WREGOE RA-W C15-18,,,,4,,
+30/12/2018 02:57:42,JohnTAss,Taurus Dark Region DB-X d1-26,6 E A,https://i.imgur.com/mFy538C.png,Small Reposing Leviathans,3,No,Yes,WREGOE RA-W C15-18,WREDGUIA VX-L D7-80,HIP 23269,,,,4,,
+30/12/2018 03:17:19,JohnTAss,Wredguia VX-L d7-80,5 F,https://i.imgur.com/bo8Lnkw.png,Small Reposing Leviathans,3,No,Yes,TAURUS DARK REGION DB-X D1-26,HIP 23269,COL 285 SECTOR ZZ-Y D22,,,,4,,
+30/12/2018 03:32:35,JohnTAss,Wredguia UB-W c15-0,1 A,https://i.imgur.com/O4adAB6.png,Small Reposing Leviathans,4,Yes,Yes,WREDGUIA VX-L D7-80,PLEIADES SECTOR BG-X C1-14,MEL 22 SECTOR IS-S C4-6,,,,6,,
+30/12/2018 03:52:14,JohnTAss,HIP 19026,B 1 C,https://i.imgur.com/Vg6TWMz.png,No Leviathans,0,No,No,,,,,,,0,,
+30/12/2018 04:10:09,JohnTAss,Mel 22 Sector IS-S c4-6,ABC 2 B,https://i.imgur.com/EU8zGZc.png,Small Reposing Leviathans,4,Yes,Yes,,,,EE overwrote messages,,,3,,
+30/12/2018 11:25:55,JohnTAss,HIP 22375,3 A,https://i.imgur.com/rTGEOUv.jpg,Large upright leviathans,4,Yes,Yes,,,,EE overwrote messages,,,4,,
+30/12/2018 11:42:58,JohnTAss,HIP 21373,2 F,https://i.imgur.com/QtNfh6w.jpg,Large upright leviathans,3,No,Yes,,,,EE overwrote messages,,,4,,
+30/12/2018 12:01:20,JohnTAss,HIP 20989,3 C,https://i.imgur.com/wYpGSP4.png,Large upright leviathans,3,No,Yes,HIP 21373,TAURUS DARK REGION DL-Y D54,TAURUS DARK REGION BQ-Y C12,,,,4,,
+30/12/2018 12:23:03,JohnTAss,Taurus Dark Region DL-Y d54,ABC 1 C,https://i.imgur.com/XOzgPu5.png,Small Reposing Leviathans,3,No,Yes,HIP 20989,TAURUS DARK REGION BQ-Y C12,HIP 21373,,,,4,,
+30/12/2018 12:42:12,JohnTAss,HIP 20644,A 2 D,https://i.imgur.com/ihvcCI4.png,Large upright leviathans,4,Yes,Yes,TAURUS DARK REGION BV-Y C11,HIND SECTOR GR-W D1-13,TAURUS DARK REGION QN-T B3-0,,,,5,,
+30/12/2018 13:11:05,JohnTAss,Taurus Dark Region QN-T b3-0,1 B,https://i.imgur.com/V398Yjg.png,Large upright leviathans,4,Yes,Yes,HIP 20644,HIP 19665,TAURUS DARK REGION BV-Y C11,,,,4,,
+30/12/2018 13:28:09,JohnTAss,Taurus Dark Region BV-Y c11,8 A,https://i.imgur.com/hWcvyZr.png,Large upright leviathans,4,Yes,Yes,HIP 20644,TAURUS DARK REGION QN-T B3-0,TAURUS DARK REGION CQ-Y D31,,,,6,,
+30/12/2018 13:46:43,JohnTAss,Taurus Dark Region CQ-Y d31,3 C,https://i.imgur.com/Va7rrOm.png,Large upright leviathans,3,No,Yes,PLEIADES SECTOR ZZ-Y C14,HIP 22375,TAURUS DARK REGION BV-Y C11,,,,4,,
+30/12/2018 14:02:30,JohnTAss,Pleiades Sector AV-Y c7,1 B,https://i.imgur.com/Vm9CXYf.png,Large upright leviathans,4,Yes,Yes,,,,EE overwrote messages,,,4,,
+30/12/2018 14:19:55,JohnTAss,Taurus Dark Region QO-Q b5-0,B 2 A,https://i.imgur.com/6aYAjMx.png,Large upright leviathans,4,Yes,Yes,PLEIADES SECTOR AV-Y C7,HIP 22375,TAURUS DARK REGION FW-W C1-3,,,,3,,
+31/12/2018 01:17:39,Revina Hardon,HIP 17694,B 7 F,,Large upright leviathans,0,None That I saw,No,,,,,,,2,,
+04/01/2019 00:36:38,LCU No Fool Like One,Pleiades Sector OD-S b4-1,2 A,https://i.imgur.com/Y5Dcmmm.jpg,Large upright leviathans,3,No,Yes,Magec,,,Only two messages. EE Overwrote,,,4,,
+06/01/2019 00:17:11,LCU No Fool Like One,HIP 16704,5 A,https://i.imgur.com/lOuNLNI.png,Large upright leviathans,4,One door was blocked,Yes,,,,,,,4,,
+10/01/2019 13:17:15,Joseph Wood,HIP 17862,11 E,,Large upright leviathans,,,No,,,,,,,1,,
+10/01/2019 14:09:41,Joseph Wood,HIP 17694,B 7 F,,Large upright leviathans,4,,,,,,,,,2,,
+12/01/2019 05:50:24,ByteSturm,HIP 17862,11 E,https://i.imgur.com/hP0M2ny.png,Small Reposing Leviathans,4,"No, everything is caved in.",No,,,,"It's hard to see,
+Everything's black,
+I'm starting to worry,
+It's time to head back.",,,1,,
+12/01/2019 06:23:13,ByteSturm,HIP 17694,B 7 F,https://i.imgur.com/AfdLaYq.png,Small Reposing Leviathans,4,No.,No,,,,"Another site
+Without a bite.
+It makes me frown,
+But at least that's another one down.",,,2,,
+12/01/2019 06:49:45,DrunkEng1n33r,HIP 17694,B 7 F,https://i.imgur.com/FN9dnXb.png,Small Reposing Leviathans,2,No.,No,,,,"Another site
+Without a bite.
+It gives me a frown,
+But at least that's one more stop down.",,,2,,
+12/01/2019 06:51:01,DrunkEng1n33r,HIP 17862,11 E,https://i.imgur.com/3Gpwkq0.png,Small Reposing Leviathans,2,No.,No,,,,"It's hard to see,
+Everything's black.
+I'm starting to worry,
+It's time to head back.",,,1,,
+13/01/2019 23:24:57,bluecrash,delphi,5a,,mix,2,nothing that opened or closed just entryways,Yes,probe,link,sensor,"there were stars below the map much like the PSR stars that are out of reach of our galaxy camera, but you can see them briefly if you type psr in search in galaxy mode. So i was below a pancake of start (much like how SAG A seems flat if you zoom away from it.)",,,4,,
+22/01/2019 18:17:53,DrunkEng1n33r,HIP 17862,11 E,https://i.imgur.com/YTXvcvp.jpg,Small Reposing Leviathans,2,No.,No,,,,"Last time I messed it up,
+So I had to revisit this site.
+Now the information's all correct,
+Everything's alright.",,,1,,
+22/01/2019 18:43:21,DrunkEng1n33r,HIP 17694,B 7 F,https://i.imgur.com/QrEjZiF.jpg,Small Reposing Leviathans,2,No.,No,,,,"Hurried on over to the next mistake,
+Fixed it real good with new info.
+Gotta keep on going I know what's at stake,
+There's no more taking it slow, I gotta go!",,,2,,
+22/01/2019 20:13:12,DrunkEng1n33r,Hind Sector GR-W d1-13,8 H,https://i.imgur.com/goJhVSW.png,Small Reposing Leviathans,2,No.,No,,,,"Another site found,
+Another system down.
+Off to the next system,
+Off to where more stars glisten.",,,2,,
+22/01/2019 21:13:15,DrunkEng1n33r,Pleiades Sector OS-U c2-7,4 A,https://i.imgur.com/moC0a1Z.jpg,No Leviathans,2,No.,No,,,,"Took a while to count the rocks.
+Tried to shine a light,
+But that only gave the scavengers a fright.
+So I went back to counting rocks.",,,0,,
+22/01/2019 21:54:03,DrunkEng1n33r,Mel 22 Sector AX-A b14-1,1 B,https://i.imgur.com/MYwCeTh.jpg,No Leviathans,2,No.,No,,,,"Nothing new,
+Nothing to see.
+Nothing new
+Here for me.",,,0,,
+06/02/2019 00:09:41,Teronis1746,Pleiades Sector GW-W d1-54,2 C,https://i.imgur.com/DOWEyiP.png,Large upright leviathans,3,"Yes, outer ring",Yes,PLEIADES SECTOR OY-R C4-19,PLEIADES SECTOR LS-T C3-2,HIP 22347,"The exterior of the device's main structure is very green. Like, algae green. ",,,4,,
+07/02/2019 02:14:07,Teronis1746,Pleiades Sector MC-V c2-0,1 A,https://i.imgur.com/9U2dyoI.png,Small Reposing Leviathans,3,No,Yes,HIP 20785 (All 3 consistent with spreadsheet),PLEIADES SECTOR MC-V C2-8,SYNUEFE UZ-X C17-10,Bright orange colored sand around inner structure's exterior.,,,4,,
+14/02/2019 01:17:16,LCU No Fool Like One,Mel 22 Sector JC-V d2-14,A 4 A,https://i.imgur.com/pVU1Zz5.jpg,Large upright leviathans,,,,,,,Lots if thargoid links in the contacts screen,3,,6,,
+18/02/2019 00:14:33,LCU No Fool Like One,Mel 22 Sector IO-G b11-1,1 A,https://i.imgur.com/fqQXmSf.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,4,,4,,
+18/02/2019 00:17:02,LCU No Fool Like One,Mel 22 Sector OY-R c4-5,2 A,https://i.imgur.com/cCi3A3L.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,3,,5,,
+18/02/2019 21:30:13,LCU No Fool Like One,Mel 22 Sector JC-V d2-31,A 16 B,https://i.imgur.com/3d15tbE.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,3,,6,,
+18/02/2019 21:54:41,LCU No Fool Like One,Mel 22 Sector HH-V d2-37,AB 1 A,https://i.imgur.com/BtxB15d.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,4,,3,,
+18/02/2019 22:23:13,LCU No Fool Like One,Mel 22 Sector HH-V d2-53,1 B,https://i.imgur.com/c1j509o.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,2,,3,,
+18/02/2019 23:34:17,LCU No Fool Like One,Mel 22 Sector FH-U c3-9,A 1 A,https://i.imgur.com/EYixhTi.jpg,Large upright leviathans,,,,,,,,4,,6,,
+19/02/2019 00:01:12,LCU No Fool Like One,Taurus Dark Region MY-R b4-1,1 B,https://i.imgur.com/7Yp6ZWg.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,4,,5,,
+19/02/2019 17:21:19,JohnTAss,Pleiades Sector EB-X c1-2,ABC 3 C,https://i.imgur.com/Y9KLGBT.jpg,,,,,,,,,,,4,,
+19/02/2019 17:40:11,JohnTAss,HIP 20859,A 1 A,https://i.imgur.com/co2Clug.jpg,,,,,,,,,1,,4,,
+19/02/2019 17:51:33,JohnTAss,Pleiades Sector EL-Y d23,ABCD 1 A,https://i.imgur.com/wQDWi3T.jpg,,,,,,,,,,,5,,
+19/02/2019 18:01:40,JohnTAss,Pleiades Sector ZZ-Y c14,A 1 A,https://i.imgur.com/nxkBjvH.jpg,,,,,,,,,,,6,,
+19/02/2019 18:14:22,JohnTAss,Taurus Dark Region CQ-Y d36,7 A,https://i.imgur.com/5csd93b.jpg,,,,,,,,,,,4,,
+20/02/2019 00:19:53,LCU No Fool Like One,Taurus Dark Region CL-Y d53,A 4,https://i.imgur.com/gcEsVLE.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,3,,3,,
+20/02/2019 00:23:39,LCU No Fool Like One,Mel 22 Sector DB-X d1-46,A 8 A,https://i.imgur.com/q6RKIex.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,4,,4,,
+20/02/2019 00:26:52,LCU No Fool Like One,Mel 22 Sector JX-T c3-9,6 A,https://i.imgur.com/yrwWjZj.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,5,,
+20/02/2019 00:29:17,LCU No Fool Like One,HIP 15889,11 B,https://i.imgur.com/x5Yx9kn.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,5,,4,,
+20/02/2019 00:32:04,LCU No Fool Like One,HIP 16795,A 10 F,https://i.imgur.com/iymNZum.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,5,,3,,
+20/02/2019 19:09:37,JohnTAss,HIP 22743,B 1 A,https://i.imgur.com/zedVYzl.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,,,6,,
+20/02/2019 19:23:38,JohnTAss,Taurus Dark Region JH-V c2-9,AB 1 A,https://i.imgur.com/MGV3EzR.jpg,Small Reposing Leviathans,,,,,,,,,,4,,
+20/02/2019 19:37:11,JohnTAss,HIP 22334,1 D,https://i.imgur.com/hgnzqiH.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,,,5,,
+20/02/2019 19:49:56,JohnTAss,HIP 21348,A 2 B,https://i.imgur.com/sw7VqZr.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,,,4,,
+20/02/2019 20:05:25,JohnTAss,HIP 22118,B 3 A,https://i.imgur.com/2aE9cgu.jpg,Small Reposing Leviathans,,,,,,,,,,4,,
+21/02/2019 18:33:41,JohnTAss,Synuefe HZ-M d8-69,B 7 F,https://i.imgur.com/v6xnMdz.jpg,Small Reposing Leviathans,,,,,,,,5,,4,,
+21/02/2019 18:47:56,JohnTAss,Pleiades Sector KH-V c2-7,7 F,https://i.imgur.com/6HOh3Ct.jpg,Small Reposing Leviathans,,,,,,,Not sure if these are small leviathans or none,,,0,,
+21/02/2019 19:00:14,JohnTAss,Pleiades Sector IM-V c2-16,ABC 2 A,https://i.imgur.com/jU726vr.png,Large upright leviathans,,,,,,,,,,1,,
+21/02/2019 19:14:28,JohnTAss,Pleiades Sector GW-W d1-65,10 F,https://i.imgur.com/bKvAQZd.jpg,Small Reposing Leviathans,,,,,,,"There are either a few small, or none here depending on what you are counting",,,0,,
+21/02/2019 23:48:40,LCU No Fool Like One,Taurus Dark Region FW-W c1-3,1 A,https://i.imgur.com/GdjsjO9.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,4,,5,,
+21/02/2019 23:52:22,LCU No Fool Like One,Taurus Dark Region BQ-Y c12,2 A,https://i.imgur.com/wevcLXH.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,5,,
+21/02/2019 23:54:45,LCU No Fool Like One,Hind Sector GR-W d1-13,8 H,https://i.imgur.com/VvaCGyY.jpg,Small Reposing Leviathans,,,,,,,,5,,2,,
+21/02/2019 23:56:47,,HIP 19665,8 B,https://i.imgur.com/ZvnIcqD.jpg,Large upright leviathans,,,,,,,,1,,3,,
+25/02/2019 11:22:40,Septica,HIP 17862,11 E,,Small Reposing Leviathans,0,No,No,,,,,4,,1,,
+25/02/2019 12:33:23,,HIP 17694,B 7 F,,Large upright leviathans,0,,No,,,,,3,,2,,
+25/02/2019 22:20:04,LCU No Fool Like One,Pleiades Sector DQ-Y c4,6 A,https://i.imgur.com/AFOnuGr.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,4,,5,,
+25/02/2019 23:46:18,LCU No Fool Like One,Pleiades Sector GG-Y d33,3 A,https://i.imgur.com/1hCm8ke.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,6,,
+26/02/2019 00:11:28,LCU No Fool Like One,Struve's Lost Sector OI-T c3-3,2 B,https://i.imgur.com/OGhdnee.jpg,,,,,,,,,,,4,,
+27/02/2019 23:37:48,LCU No Fool Like One,HIP 16985,A 4 E,https://i.imgur.com/SsjRPGn.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,6,,
+02/03/2019 23:12:56,ClusterNuke63,OUTOTZ ST-I D9-6,2 A,https://cdn.discordapp.com/attachments/344094711339941890/551512169074786315/image0.jpg,,,,,,,,,5,,2,,
+04/03/2019 09:39:31,LCU No Fool Like One,Mel 22 Sector MX-U d2-14,AB 1 A,https://i.imgur.com/2BqUCpB.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,,,3,,
+04/03/2019 09:42:28,LCU No Fool Like One,Mel 22 Sector TT-R c4-2,3 A,https://i.imgur.com/9BE9Gj9.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,6,,
+04/03/2019 09:45:05,LCU No Fool Like One,Mel 22 Sector KC-V d2-28,1 C,https://i.imgur.com/z4gig2N.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,3,,5,,
+04/03/2019 09:48:53,LCU No Fool Like One,Mel 22 Sector NX-U d2-31,3 A,https://i.imgur.com/R5HzHJC.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,3,,5,,
+13/03/2019 20:10:03,Crimshadow2,HIP 20964, 3 B,https://cdn.discordapp.com/attachments/555431538745933834/555435376622436352/unknown.png,Small Reposing Leviathans,0,N/A,No,,,,I heard pretty average joke concerning Orion's Belt. Its about 3 stars.,4,,1,,
+13/03/2019 22:57:01,LCU No Fool Like One,COL 285 SECTOR CV-Y D57, AB 4 A,https://i.imgur.com/wimzXiu.jpg,No Leviathans,,,,,,,,5,,0,,
+17/03/2019 20:17:48,LCU No Fool Like One,HIP 18909, A 4 E A,https://i.imgur.com/JvvGxnn.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,4,,
+17/03/2019 21:05:26,LCU No Fool Like One,PLEIADES SECTOR MX-U D2-54, 4 E,https://i.imgur.com/HE223UA.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,4,,
+17/03/2019 23:18:24,LCU No Fool Like One,PLEIADES SECTOR GW-W D1-54, 2 C,https://i.imgur.com/S99VdFU.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,4,,
+18/03/2019 00:12:07,LCU No Fool Like One,PLEIADES SECTOR OY-R C4-19, A 3 A,https://i.imgur.com/n2fV2gr.jpg,Large upright leviathans,,,,,,,,1,,4,,
+18/03/2019 14:34:49,LCU No Fool Like One,PLEIADES SECTOR VK-N B7-0, C 1,https://i.imgur.com/9dR4BqP.jpg,No Leviathans,,,,,,,,1,,0,,
+19/03/2019 00:01:25,LCU No Fool Like One,HIP 17837, A 4 B,https://i.imgur.com/ozI0nbx.jpg,Large upright leviathans,,,,,,,,1,,4,,
+19/03/2019 00:23:29,LCU No Fool Like One,HIP 16440, 1 E,https://i.imgur.com/cSyroWv.jpg,,,,,,,,,1,,4,,
+20/03/2019 07:36:36,Teronis1746,Pleiades Sector JM-W d1-52,2 A,,Large upright leviathans,4,Yes,Yes,12 Trianguli – Taylor Keep INRA base,UGP 145 13 Sep. 3304 (2018),None - altered by EE,"8 spires with Meta-Alloys surrounding structure. Bright orange sand around main site. Largest Leviathins I have seen. Quite a lot of ""eggs"" inside"". ",3,None,4,,
+23/03/2019 00:41:27,LCU No Fool Like One,PLEIADES SECTOR LN-T C3-11, 9 A,https://i.imgur.com/QnH09Ox.jpg,No Leviathans,,,,,,,,1,,0,,
+23/03/2019 01:11:11,LCU No Fool Like One,PLEIADES SECTOR JS-T C3-17, 1 A,https://i.imgur.com/mLIcanp.jpg,No Leviathans,,,,,,,,4,,0,,
+23/03/2019 02:13:18,LCU No Fool Like One,ARIES DARK REGION DB-X D1-63, A 7 A,https://i.imgur.com/Nbgju7s.jpg,No Leviathans,,,,,,,,1,,0,,
+23/03/2019 20:28:07,LCU No Fool Like One,ARIES DARK REGION DB-X D1-64, 4 A,https://i.imgur.com/iYVk9w1.jpg,Small Reposing Leviathans,,,,,,,This could be two leviathans. I think we need a daylight image,1,,2,,
+23/03/2019 20:50:58,LCU No Fool Like One,COL 285 SECTOR RI-R B5-3, 8 B,https://i.imgur.com/7kbe6hS.jpg,Small Reposing Leviathans,,,,,,,,4,,1,,
+23/03/2019 21:02:48,LCU No Fool Like One,HIP 16692, 4 C,https://i.imgur.com/b16OttG.jpg,Small Reposing Leviathans,,,,,,,,4,,1,,
+23/03/2019 21:41:52,LCU No Fool Like One,PLEIADES SECTOR OY-R C4-19, A 3 A,https://i.imgur.com/LKu3zvK.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,4,,
+23/03/2019 22:15:03,LCU No Fool Like One,HYADES SECTOR AQ-Y D81, AB 3 C,https://i.imgur.com/zdb8yN6.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,5,,4,,
+23/03/2019 22:25:33,LCU No Fool Like One,HYADES SECTOR ZU-Y C16, 6 B,https://i.imgur.com/WAPogtF.jpg,Small Reposing Leviathans,,,,,,,,1,,2,,
+23/03/2019 22:39:59,LCU No Fool Like One,HYADES SECTOR AQ-Y D93, 2 B,https://i.imgur.com/GZpB9Dg.jpg,No Leviathans,,,,,,,,2,,0,,
+23/03/2019 22:46:38,Petrenus,HIP 20964, 3 B,https://photos.google.com/share/AF1QipM-mwx2MQzYnmFxT67xsXVe34vS6o1ZJnYxPTApz-3SccDKBDNODpXY1EcKKqXW3g/photo/AF1QipNO-NHH4R6_gDihpL4Y7Mzo9oSe5q-FBLUQVLxY?key=UnlTY3FyRnFwU1RHZy1wMGNVeVNTWi1zcFRHRzJB,,,,No,,,,I did not have a probe with me to try the door but it looked blocked. One leviathan structure that looks half burried as far as I can tell. Scavengers are active on the leviathan part but it seems only there.,2,,1,,https://photos.app.goo.gl/AwipDVF9iDaBrzD78
+24/03/2019 13:29:59,Petrenus,HYADES SECTOR XO-A C15, 2 A,https://lh3.googleusercontent.com/Dr62qyE4SA77CFwEjhRdTJfAgwTKzr3aHUiSVTjIUSNkdBXwwL-bu4t_09d_n_l8ECikdPQjXud-zyRU8r-BZHLHiG6ECWsHoa26D2mdzASBJ8tMYQk82mNVZwegXtIWxPX3cTxbxhN3TVHGQC3U8d_CgO0IZSuttLbpHPTJRwloyiUNJdJKx8NmPPsOu3iBmyBaL5N2oPcDvmTVcQn9-RK2Qcl9R-bcgsZ6EAcx8DwvA4YCXbCjDvDL2rGvLjZWzMZ74XKjwrv0ub6VvQUnO9fieEv2xRJTgiMw5gXWJYaKEKCsqEe5FoLZMXhAL8QVdLfaO3FGRzZWlFPpgxf9n8frdSVQZ-KTjwqaMl-pOBNbydn0mu8KFOcS8dxXPzVC9kRTPjISWcoTwvdpYO-P522AfqOIgvgYDiR_t6763dicUDzxOvLConkDh1VxN2fzJQeQZ2rqx9hzj-UhKIRwGVgMFMvw-nHY0BIszgoy9LS-Y09nQ8GPFxkKjzt16EcCRFsP2sLvFX0MKw-ueqOVldWrbxt-A2Ga1-Fp5ARWH1CaEpIwkFJNhVwh3R6uHfXVHzH4CgoBek1TCW7gXlfbI62VDCa7t2qgPJAGeJkduGOLbGQVnYe3gUyTOxPxtFB289mtKOA5oOJp7JiXLexnVEeYWCvzk_Qm8xtByyrlyU7zLZvvmR-04BVO6jhvFLyHRGJT70xwHaGeKXFjckYUusI=w1487-h836-no,Small Reposing Leviathans,0,,,,,,No probes with me to open the doors. I really need to get access to Palin.,4,,1,,https://photos.app.goo.gl/vHgZwHSoMGbRpUP97
+25/03/2019 23:34:48,Vallysa,HYADES SECTOR PC-V B2-3, 2 A,https://i.imgur.com/qTWBWmY.jpg,Large upright leviathans,,,,,,,"Lots of scavengers running around, probably plenty of meta-alloys to harvest. #badpoetry",4,,1,,
+25/03/2019 23:54:42,LCU No Fool Like One,COL 285 SECTOR CV-Y D71, 5 D,https://i.imgur.com/fqTc5I6.jpg,,,,,,,,,1,,2,,
+28/03/2019 01:46:11,OverloadRJ45,HIP 15134, 3 B,https://drive.google.com/file/d/1a9Tu25vl83x3hgSPXnXKp153e5j5qmg_/view?usp=sharing,Small Reposing Leviathans,,,,,,,"Space is big
+Space is dark
+It's hard to find
+A place to park.
+
+There was a lot more activity at this site than I expected from the term ""Inactive""; I killed around two dozen of the scavengers.",4,,2,,
+28/03/2019 17:50:20,Capitan Radz,HIP 19266, 6 A,https://i.imgur.com/VTnyxWy.png,,2,,No,,,,,5,Accesible by VRS,4,,
+28/03/2019 21:58:43,Wanakamura,HIP 15134, 3 B,https://photos.smugmug.com/photos/i-HFbW2tD/0/94724795/4K/i-HFbW2tD-4K.jpg,"Large upright leviathans, Small Reposing Leviathans",3,,No,,,,more photos https://www.krochmal.eu/EliteDangerous/Hip15134-B3/n-gkpdD2/i-HFbW2tD/A,4,yes,2,,
+28/03/2019 22:32:52,Wanakamura,ARIES DARK REGION MS-T C3-15, 1 A,https://photos.smugmug.com/photos/i-TMGrm62/0/f112fb37/5K/i-TMGrm62-5K.jpg,"Large upright leviathans, Small Reposing Leviathans",3,,No,,,,,4,all blocked,2,,
+28/03/2019 23:32:24,Cordon Bleu,HYADES SECTOR PC-V B2-3, 2 A,https://drive.google.com/drive/folders/1Kyyz7XDrqPqjWTLUqO8m55HTDBzUZe6c?usp=sharing,,,,,,,,I dont understand what Leviathans are. Please view the pictures.,2,,1,,
+29/03/2019 14:10:52,Cordon Bleu,HYADES SECTOR PC-V B2-3, 2 A,https://drive.google.com/drive/folders/1Kyyz7XDrqPqjWTLUqO8m55HTDBzUZe6c?usp=sharing,,,,,,,,I have added a picture of the site in daylight. Hope they are useful.,4,,1,,
+29/03/2019 18:26:01,Maylor Rom,SYNUEFE JU-M D8-50, 5 A,https://i.imgur.com/FKKhF9m.jpg,Small Reposing Leviathans,0,Unknown,,,,,Unable to enter due to no Alien Artifacts on board.,5,Unknown,3,,
+29/03/2019 19:26:57,Misterbowwow,TAURUS DARK REGION BV-Y C11, 8 A,https://imgur.com/a/sCs6Cwq,Large upright leviathans,,,,,,,,4,,6,,
+29/03/2019 19:35:05,Wanakamura,DELPHI, 5 A,https://photos.smugmug.com/photos/i-Nh4673b/0/16bd639b/5K/i-Nh4673b-5K.jpg,,,,,,,,,3,,4,,
+30/03/2019 10:51:47,Xeno,Delphi,5 A,https://twitter.com/aditbharadwaj/status/1111912897293107200,"Large upright leviathans, Small Reposing Leviathans",,,,,,,I didn't go inside because I didn't had a sensor/probe,1,,4,,
+30/03/2019 18:47:02,Shoja Kamalu,ARIES DARK REGION NN-T C3-12, 1 D,https://cdn.discordapp.com/attachments/553431774026268676/553431858847809536/Elite_Dangerous_Screenshot_2019.03.07_-_22.36.12.53.png,,,,,,,,,1,,1,,
+30/03/2019 19:06:13,Shoja Kamalu,HIP 15134,3 B,https://cdn.discordapp.com/attachments/553431774026268676/553921696286113792/HIP_15134_3_b_00003.png,,,,,,,,,1,,2,,
+30/03/2019 22:37:48,Shoja Kamalu,ARIES DARK REGION MS-T C3-15,1 A,https://cdn.discordapp.com/attachments/553431774026268676/553938515683966976/Aries_Dark_Region_MS-T_c3-15_1_a_00001.png,,,,,,,,,1,,2,,
+30/03/2019 22:39:29,Shoja Kamalu,HIP 14908,A 2 A,https://cdn.discordapp.com/attachments/553431774026268676/553954150568689674/HIP_14908_A_2_a_00003.png,,,,,,,,,3,,2,,
+30/03/2019 22:40:11,Shoja Kamalu,HIP 11457,1 C,https://cdn.discordapp.com/attachments/553431774026268676/553993464354701358/HIP_11457_1_c_00001.png,No Leviathans,,,,,,,,1,,0,,
+30/03/2019 22:40:52,Shoja Kamalu,HIP 11700,1 A,https://cdn.discordapp.com/attachments/553431774026268676/554043575743283200/HIP_11700_1_a_00001.png,Large upright leviathans,,,,,,,,1,,4,,
+30/03/2019 22:42:24,Shoja Kamalu,ARIES DARK REGION MX-U C2-8,A 7 A,https://cdn.discordapp.com/attachments/553431774026268676/554046710519693313/Aries_Dark_Region_MX-U_c2-8_A_7_a_00003.png,,,,,,,,,2,,4,,
+30/03/2019 22:50:36,LCU No Fool Like One,MEL 22 SECTOR AX-A B14-1,1 B,https://cdn.discordapp.com/attachments/296717350575800320/561683376742989844/HighRes_Mel_22_Sector_AX-A_b14-1__1_b_LCU_No_Fool_Like_One_00001.png,,,,,,,,,5,,0,,
+30/03/2019 22:55:10,LCU No Fool Like One,MEL 22 SECTOR JC-V D2-19,4 B,https://i.imgur.com/bCG54be.jpg,,,,,,,,,5,,3,,
+30/03/2019 22:58:24,LCU No Fool Like One,MEL 22 SECTOR JC-V D2-41,A 1 A,https://i.imgur.com/JpG7EB8.jpg,,,,,,,,,1,,4,,
+30/03/2019 23:00:35,LCU No Fool Like One,MEL 22 SECTOR SK-E B12-1,2,https://i.imgur.com/TPWuxao.jpg,,,,,,,,,4,,3,,
+30/03/2019 23:02:36,LCU No Fool Like One,HIP 15746,AB 1 B,https://i.imgur.com/olUxYCd.jpg,,,,,,,,,1,,6,,
+30/03/2019 23:04:30,LCU No Fool Like One,PLEIADES SECTOR EC-U B3-0,4 A,https://i.imgur.com/mV0O1Fx.jpg,,,,,,,,,,,4,,
+30/03/2019 23:06:29,LCU No Fool Like One,PLEIADES SECTOR KT-Q B5-3,5 A,https://i.imgur.com/R2VPqTb.png,,,,,,,,,1,,5,,
+30/03/2019 23:08:15,LCU No Fool Like One,PLEIADES SECTOR HH-V C2-13,A 1 C,https://i.imgur.com/F8PY7ZT.jpg,,,,,,,,,1,,4,,
+30/03/2019 23:14:56,LCU No Fool Like One,PLEIADES SECTOR JC-V C2-9,1 A,https://i.imgur.com/hQtJKaX.jpg,,,,,,,,,1,,4,,
+30/03/2019 23:16:43,LCU No Fool Like One,PLEIADES SECTOR LX-U C2-2,AB 4 A,https://i.imgur.com/ofFwPM5.jpg,,,,,,,,,1,,4,,
+30/03/2019 23:18:29,LCU No Fool Like One,PLEIADES SECTOR OS-U C2-7,4 A,https://i.imgur.com/gyA5IWl.jpg,,,,,,,,,1,,0,,
+30/03/2019 23:20:26,LCU No Fool Like One,PLEIADES SECTOR OS-U C2-6,A 5 B,https://i.imgur.com/xN2QG8y.jpg,,,,,,,,,1,,4,,
+30/03/2019 23:22:52,LCU No Fool Like One,MEL 22 SECTOR CB-O C6-4,3 A,https://i.imgur.com/fbMd2ef.jpg,,,,,,,,,1,,4,,
+30/03/2019 23:24:45,LCU No Fool Like One,HIP 14909,2 A,https://i.imgur.com/U32ENc4.jpg,,,,,,,,,5,,4,,
+30/03/2019 23:26:26,LCU No Fool Like One,MEL 22 SECTOR ZU-P C5-1,4 A,https://i.imgur.com/WHhlyEJ.jpg,,,,,,,,,1,,4,,
+30/03/2019 23:28:42,LCU No Fool Like One,MEL 22 SECTOR NX-U D2-27,5 A,https://i.imgur.com/mskG2su.jpg,,,,,,,,,1,,4,,
+01/04/2019 10:38:37,LCU No Fool Like One,HIP 15134, 3 B,https://i.imgur.com/G9W4hmw.jpg,,,,,,,,,1,,2,,
+01/04/2019 10:47:44,LCU No Fool Like One,ARIES DARK REGION MS-T C3-15, 1 A,https://i.imgur.com/cqR5tW4.jpg,,,,,,,,,3,,2,,
+01/04/2019 10:59:21,LCU No Fool Like One,HIP 14908, A 2 A,https://i.imgur.com/vjtbqSD.jpg,,,,,,,,,1,,2,,
+01/04/2019 12:13:46,LCU No Fool Like One,ARIES DARK REGION HR-W D1-34, 2 A,https://i.imgur.com/UwtacYq.jpg,,,,,,,,,3,,4,,
+01/04/2019 12:56:09,LCU No Fool Like One,ARIES DARK REGION VT-R B4-0, A 6,https://i.imgur.com/x1dau24.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,2,,4,,
+02/04/2019 12:42:52,LCU No Fool Like One,PLEIADES SECTOR OD-S B4-1, 2 A,https://i.imgur.com/mx2msNE.jpg,,,,,,,,,2,,4,,
+02/04/2019 13:06:15,LCU No Fool Like One,PLEIADES SECTOR IH-V C2-3, 1 B,https://i.imgur.com/yJ0zA5r.jpg,,,,,,,,,1,,4,,
+02/04/2019 14:58:19,LCU No Fool Like One,PLEIADES SECTOR HH-V C2-6, 2 A,https://i.imgur.com/ernr6Hw.jpg,,,,,,,,,5,,4,,
+02/04/2019 15:21:24,LCU No Fool Like One,PLEIADES SECTOR EB-X C1-2, ABC 3 C,https://i.imgur.com/KK1eaZq.jpg,,,,,,,,,2,,4,,
+07/04/2019 18:20:11,LCU No Fool Like One,PLEIADES SECTOR UZ-X B1-2, A 1 C,https://i.imgur.com/0r2CDIi.jpg,No Leviathans,,,,,,,,4,,0,,
+07/04/2019 20:35:06,LCU No Fool Like One,PLEIADES SECTOR BG-X C1-10, 14 A,https://i.imgur.com/L6lAQA3.jpg,Large upright leviathans,,,,,,,,1,,3,,
+07/04/2019 22:02:45,LCU No Fool Like One,PLEIADES SECTOR BG-X C1-14, 5 D,https://i.imgur.com/cfYwSDY.jpg,Large upright leviathans,,,,,,,,1,,4,,
+07/04/2019 22:30:25,LCU No Fool Like One,MEL 22 SECTOR FM-V D2-38, A 3 A,https://i.imgur.com/wQWzQf8.jpg,Large upright leviathans,,,,,,,,1,,3,,
+07/04/2019 22:45:30,LCU No Fool Like One,MEL 22 SECTOR ZM-H B11-3, B 3,https://i.imgur.com/l7SYcJX.jpg,Large upright leviathans,,,,,,,,4,,5,,
+07/04/2019 23:00:13,LCU No Fool Like One,MEL 22 SECTOR NT-Q C5-11, 8 A,https://i.imgur.com/lPUL2ee.jpg,,,,,,,,,1,,4,,
+08/04/2019 01:15:29,LCU No Fool Like One,HIP 20989, 3 C,https://i.imgur.com/DJxgd3s.jpg,Large upright leviathans,,,,,,,,1,,4,,
+08/04/2019 09:05:36,I C Aleinns,DELPHI, 5 A,https://i.imgur.com/RdHwd5Z.png,Small Reposing Leviathans,,,,,,,,1,,4,,
+09/04/2019 19:34:20,Alkibiades,DELPHI, 5 A,https://i.imgur.com/4gkhQMN.png,,,,,,,,,4,,4,,
+09/04/2019 20:51:18,ElectricNacho,HIP 18909, A 4 E A,https://steamuserimages-a.akamaihd.net/ugc/963116367109735678/466292D42965D8EC242A05B9A651ECED362AE8CF/,Large upright leviathans,,,,,,,,3,,3,,
+09/04/2019 21:10:23,ElectricNacho,ARIES DARK REGION GW-W D1-48, 6 A,https://steamuserimages-a.akamaihd.net/ugc/963116367109796802/B3130FF2A7B6D86E4C5DC0FEFC570E0C3347F641/,No Leviathans,,,,,,,,4,,0,,
+09/04/2019 21:19:56,ElectricNacho,ARIES DARK REGION IM-V C2-5, 12 A,https://steamuserimages-a.akamaihd.net/ugc/963116367109826677/BA7FE8E6838D45714EAE17C27E00FA3CF2947C18/,Large upright leviathans,,,,,,,,3,,1,,
+09/04/2019 21:59:39,ElectricNacho,HIP 15134, 3 B,https://steamuserimages-a.akamaihd.net/ugc/963116367109947170/2A705C7BDCA76BC6DEAE68C791907C5B13E74CE3/,Large upright leviathans,,,,,,,,2,,2,,
+09/04/2019 22:19:43,ElectricNacho,ARIES DARK REGION MS-T C3-2, A 1 A,https://steamuserimages-a.akamaihd.net/ugc/963116367110009240/EF78DA5F78AF942FBD45E666A6ACC0D99AA5A1FE/,Large upright leviathans,,,,,,,,2,,1,,
+09/04/2019 23:34:41,ElectricNacho,MEL 22 SECTOR YU-F B11-1, AB 5 A,https://steamuserimages-a.akamaihd.net/ugc/963116367110228078/2508FFC1C263A7BC066FD7177C8739D1C8D84220/,Large upright leviathans,,,,,,,"NHSS 5 x3, NHSS 3 x1 in system",5,,5,,
+09/04/2019 23:44:19,ElectricNacho,MEL 22 SECTOR UT-R C4-4, 1 A,https://steamuserimages-a.akamaihd.net/ugc/963116367110261972/19978B349BA7A7C74B8379F2BBA293FA1BED8F87/,Large upright leviathans,,,,,,,,3,,4,,
+10/04/2019 23:15:01,Powell Oblivion,ARIES DARK REGION DB-X C1-7, A 9 A,https://i.imgur.com/XXNKCHa.jpg,,,,,,,,Thargoid Mats lying by the door,5,,4,,
+11/04/2019 10:26:40,Regemus,HYADES SECTOR PC-V B2-3, 2 A,https://i.imgur.com/hULHNj5.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,3,,1,,
+11/04/2019 13:19:52,Regemus,HIP 15134, 3 B,https://i.imgur.com/V0mHl5q.jpg,,,,,,,,,2,,2,,
+11/04/2019 21:49:31,LCU No Fool Like One,TAURUS DARK REGION DL-Y D54, ABC 1 C,https://i.imgur.com/YjMKns2.jpg,Large upright leviathans,,,,,,,,5,,4,,
+12/04/2019 00:32:00,LCU No Fool Like One,MEL 22 SECTOR IS-S C4-6, ABC 2 B,https://i.imgur.com/NURkcxc.jpg,,,,,,,,,,,3,,
+12/04/2019 00:50:29,LCU No Fool Like One,WREDGUIA UB-W C15-0, 1 A,https://i.imgur.com/6SR6JSA.jpg,,,,,,,,,2,,6,,
+12/04/2019 23:37:53,LCU No Fool Like One,MEL 22 SECTOR FM-V D2-67, 2 A,https://i.imgur.com/Qk0ZVls.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,5,,4,,
+12/04/2019 23:52:59,LCU No Fool Like One,MEL 22 SECTOR OO-Q C5-10, 7 B,https://i.imgur.com/fFaBahG.jpg,,,,,,,,,1,,5,,
+13/04/2019 00:13:01,LCU No Fool Like One,MEL 22 SECTOR LN-T D3-64, ABC 1 C,https://i.imgur.com/fAjNttl.jpg,,,,,,,,,1,,5,,
+13/04/2019 00:39:09,LCU No Fool Like One,MEL 22 SECTOR OO-Q C5-6, 1 A,https://i.imgur.com/ah9jQ4f.jpg,Large upright leviathans,,,,,,,,4,,5,,
+13/04/2019 01:40:25,ElectricNacho,PLEIADES SECTOR EB-X C1-2, ABC 3 C,https://steamuserimages-a.akamaihd.net/ugc/961990768470720463/7C205594AB196310EA01C97DB159B3A491533A7F/,Small Reposing Leviathans,,,,,,,,2,,3,,
+13/04/2019 01:55:29,ElectricNacho,PLEIADES SECTOR DQ-Y C4, 6 A,https://steamuserimages-a.akamaihd.net/ugc/961990768470767446/E4D58CDE602B72A6672948E643D9F118026E2A30/,Large upright leviathans,,,,,,,,4,,5,,
+13/04/2019 02:36:38,ElectricNacho,PLEIADES SECTOR MX-U D2-63, 1 A,https://steamuserimages-a.akamaihd.net/ugc/961990768470888181/8BD6E78F2471B207DA0D68684D9978D9184FA1C7/,No Leviathans,,,,,,,,4,,0,,
+13/04/2019 12:37:19,Ranx,HIP 17694, B 7 F,https://imgur.com/a/P2T6vch,No Leviathans,,,,,,,"Sorry for the bad picture, the exe crashed right after snapping it",2,,2,,
+14/04/2019 18:30:58,LCU No Fool Like One,ARIES DARK REGION FW-W D1-76, 4 A,https://i.imgur.com/ZaBnmpa.jpg,No Leviathans,,,,,,,,4,,0,,
+14/04/2019 18:55:10,LCU No Fool Like One,ARIES DARK REGION JS-T C3-1, 1 A,https://i.imgur.com/Z6Zbv3g.jpg,Small Reposing Leviathans,,,,,,,,1,,2,,
+14/04/2019 20:18:22,LCU No Fool Like One,COL 285 SECTOR GW-W D1-66, A 3,https://i.imgur.com/AV13Q7v.jpg,Small Reposing Leviathans,,,,,,,,4,,1,,
+14/04/2019 20:38:22,LCU No Fool Like One,HIP 12967, 1 A,https://i.imgur.com/KLH01YM.jpg,Small Reposing Leviathans,,,,,,,,1,,2,,
+14/04/2019 20:58:04,LCU No Fool Like One,HIP 11981, A 5 A,https://i.imgur.com/1VXaEfM.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,5,,4,,
+15/04/2019 22:44:24,LCU No Fool Like One,HIP 12916, 4 A,https://i.imgur.com/wXquex7.jpg,Small Reposing Leviathans,,,,,,,,1,,1,,
+15/04/2019 23:06:06,LCU No Fool Like One,ARIES DARK REGION IH-V C2-9, 6 B,https://i.imgur.com/CDQ1jVc.jpg,,,,,,,,,1,,2,,
+15/04/2019 23:35:04,LCU No Fool Like One,ARIES DARK REGION NN-T C3-12, 1 D,https://i.imgur.com/hXNKgB8.jpg,Small Reposing Leviathans,,,,,,,,4,,1,,
+16/04/2019 00:08:15,LCU No Fool Like One,HIP 11154, B 9 B,https://i.imgur.com/AL2aUma.jpg,Small Reposing Leviathans,,,,,,,,4,,2,,
+16/04/2019 16:04:22,JeronimusII,HIP 16692, 4 C,https://i.imgur.com/0NGK0iL.jpg,Small Reposing Leviathans,2,,,,,,,4,,1,,
+16/04/2019 23:55:11,LCU No Fool Like One,ARIES DARK REGION OI-T C3-8, 1 A,https://i.imgur.com/ljjl0yr.jpg,No Leviathans,,,,,,,,1,,0,,
+17/04/2019 22:10:01,LCU No Fool Like One,ARIES DARK REGION HR-W D1-54, 2 C A,https://i.imgur.com/NL63wMR.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,3,,
+17/04/2019 22:27:09,LCU No Fool Like One,ARIES DARK REGION HR-W D1-53, 7 A,https://i.imgur.com/fYyEcfA.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,4,,4,,
+17/04/2019 22:41:45,LCU No Fool Like One,ARIES DARK REGION PY-R B4-1, A 4 A,https://i.imgur.com/1c7OQWK.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,4,,4,,
+17/04/2019 23:09:27,LCU No Fool Like One,ARIES DARK REGION HR-W D1-44, B 2 A,https://i.imgur.com/LmQVoRT.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,5,,4,,
+17/04/2019 23:50:47,LCU No Fool Like One,ARIES DARK REGION AQ-Y D14, 4 A,https://i.imgur.com/b3JEYVk.jpg,No Leviathans,,,,,,,,3,,0,,
+20/04/2019 07:33:02,Bacon_,PLEIADES SECTOR OS-U C2-7, 4 A,https://i.imgur.com/ANKmroL.png,No Leviathans,,,,,,,,1,,0,,
+21/04/2019 09:39:41,Minogon,HIP 20964, 3 B,https://i.imgur.com/RKxEcxr.png,Large upright leviathans,,,,,,,,3,,1,,
+21/04/2019 18:02:01,Lybra,HIP 17694, B 7 F,https://drive.google.com/open?id=1KX4r8AO14WZw2uIuNkHv5IPWakIIXOMQ,,,,,,,,,3,,2,,
+21/04/2019 19:40:36,LCU No Fool Like One,ARIES DARK REGION DB-X C1-7, A 9 A,https://i.imgur.com/UVcTI2h.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,4,,
+21/04/2019 20:05:57,LCU No Fool Like One,ARIES DARK REGION DB-X C1-2, B 1,https://i.imgur.com/jH83rt4.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,4,,
+21/04/2019 20:23:40,LCU No Fool Like One,MEL 22 SECTOR LN-T D3-60, 5 A,https://i.imgur.com/pku4Uwj.jpg,,,,,,,,,3,,4,,
+21/04/2019 20:39:59,LCU No Fool Like One,SYNUEFAI YQ-X C17-7, 1 A,https://i.imgur.com/cHDBIS4.jpg,,,,,,,,,2,,3,,
+21/04/2019 20:51:22,LCU No Fool Like One,SYNUEFAI WV-X C17-12, 2 B,https://i.imgur.com/nHs5Fsh.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,4,,4,,
+21/04/2019 21:10:10,LCU No Fool Like One,SYNUEFAI YQ-X C17-8, 3 A,https://i.imgur.com/qqbWnuY.jpg,,,,,,,,,3,,4,,
+21/04/2019 21:22:27,LCU No Fool Like One,MEL 22 SECTOR WK-O C6-1, 5 C,https://i.imgur.com/o2GCQEE.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,5,,
+21/04/2019 22:41:09,LCU No Fool Like One,ARIES DARK REGION HR-W C1-1, 8 C,https://i.imgur.com/eyld4Nc.jpg,,,,,,,,,3,,4,,
+22/04/2019 10:56:40,LCU No Fool Like One,HIP 11700, 1 A,https://i.imgur.com/2nYQ0Q2.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,5,,4,,
+22/04/2019 23:39:06,LCU No Fool Like One,HIP 16572, A 4 E,https://i.imgur.com/UEZSvXo.jpg,Small Reposing Leviathans,,,,,,,,5,,2,,
+23/04/2019 23:24:46,LCU No Fool Like One,PLEIADES SECTOR IR-W D1-61, BC 1 A,https://i.imgur.com/zYvBIjc.jpg,,,,,,,,,3,,2,,
+23/04/2019 23:36:26,LCU No Fool Like One,PLEIADES SECTOR WE-Q B5-2, 1 A,https://i.imgur.com/8zEfqxd.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,4,,
+23/04/2019 23:49:34,LCU No Fool Like One,PLEIADES SECTOR IR-W D1-38, ABCD 1 A,https://i.imgur.com/nTtIPU0.jpg,No Leviathans,,,,,,,,5,,0,,
+25/04/2019 12:54:09,LCU No Fool Like One,PLEIADES SECTOR KH-V C2-7, 7 F,https://i.imgur.com/DajJGRe.jpg,No Leviathans,,,,,,,,5,,0,,
+25/04/2019 13:07:04,LCU No Fool Like One,PLEIADES SECTOR IM-V C2-16, ABC 2 A,https://i.imgur.com/hlpUjXw.jpg,Small Reposing Leviathans,,,,,,,,3,,1,,
+25/04/2019 13:29:00,LCU No Fool Like One,HIP 20785, 6 B,https://i.imgur.com/BDdr0Nb.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,5,,5,,
+25/04/2019 13:47:03,LCU No Fool Like One,PLEIADES SECTOR KH-V C2-1, 2 A,https://i.imgur.com/DL8lsPT.jpg,Small Reposing Leviathans,,,,,,,,1,,1,,
+25/04/2019 14:01:23,LCU No Fool Like One,PLEIADES SECTOR KH-V C2-3, 3 A,https://i.imgur.com/jEjZvG1.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,3,,4,,
+25/04/2019 14:40:35,LCU No Fool Like One,PLEIADES SECTOR IR-W D1-76, B 3 A,https://i.imgur.com/AGWlFLQ.jpg,"Large upright leviathans, Small Reposing Leviathans",,,Yes,,,,,1,,4,,
+25/04/2019 15:41:36,LCU No Fool Like One,HIP 22382, 5 E,https://i.imgur.com/1VeMTLq.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,4,,
+25/04/2019 19:03:00,LCU No Fool Like One,HIP 17044, 1 B,https://i.imgur.com/fQfNWoR.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,4,,4,,
+26/04/2019 00:21:26,LCU No Fool Like One,PLEIADES SECTOR MX-U D2-63, 1 A,https://i.imgur.com/czcTezf.jpg,No Leviathans,,,,,,,,5,,0,,
+26/04/2019 08:49:50,Tokfrans,HIP 15134, 3 B,https://i.imgur.com/atK0QGz.png,,,,,,,,,5,,2,,
+26/04/2019 21:58:11,LCU No Fool Like One,ARIES DARK REGION KH-V C2-4, 7 A,https://i.imgur.com/gAmlCpW.jpg,"Large upright leviathans, Small Reposing Leviathans",,,Yes,,,,,2,Yes,4,,
+26/04/2019 23:24:33,LCU No Fool Like One,HYADES SECTOR PC-V B2-3, 2 A,https://i.imgur.com/didUpsE.jpg,Small Reposing Leviathans,,,,,,,,1,,1,,
+27/04/2019 13:32:43,Squeesquee,HIP 16692, 4 C,https://i.imgur.com/6MtA4NQ.png,,,,,,,,,2,,1,,
+27/04/2019 15:27:24,LCU No Fool Like One,ARIES DARK REGION MS-T C3-2, A 1 A,https://i.imgur.com/YyrYxZW.jpg,Small Reposing Leviathans,,,,,,,,4,,2,,
+27/04/2019 15:41:43,LCU No Fool Like One,ARIES DARK REGION GW-W D1-48, 6 A,https://i.imgur.com/2xBTpa3.jpg,No Leviathans,,,,,,,,1,,0,,
+27/04/2019 15:55:35,LCU No Fool Like One,ARIES DARK REGION IM-V C2-5, 12 A,https://i.imgur.com/aOPuzQd.jpg,Small Reposing Leviathans,,,,,,,,1,,1,,
+27/04/2019 18:13:28,LCU No Fool Like One,HYADES SECTOR XO-A C15, 2 A,https://i.imgur.com/uTjpesL.jpg,Small Reposing Leviathans,,,,,,,,2,,1,,
+27/04/2019 19:01:28,LCU No Fool Like One,PLEIADES SECTOR LS-T C3-2, 5 A,https://i.imgur.com/EK9M3wd.jpg,"Large upright leviathans, Small Reposing Leviathans",,Yes,,,,,,1,,4,,
+27/04/2019 19:35:57,LCU No Fool Like One,PLEIADES SECTOR FW-W D1-37, 2 A,https://i.imgur.com/iF4It9q.jpg,"Large upright leviathans, Small Reposing Leviathans",,Yes,Yes,,,,,5,No,4,,
+27/04/2019 20:32:21,LCU No Fool Like One,HIP 19026, B 1 C,https://i.imgur.com/ur3r2rE.jpg,No Leviathans,,,,,,,,1,,0,,
+28/04/2019 18:26:48,Armourer,MEL 22 SECTOR FH-U C3-9, A 1 A, https://ibb.co/xh7HdMz,,0,,No,,,,,1,,6,,
+29/04/2019 22:33:29,Armourer,MEL 22 SECTOR FH-U C3-9, A 1 A,http://www.image-share.com/upload/3954/258.jpg,Large upright leviathans,4,,No,,,,Waiting for a 24 hours for good light. ))) ,4,All,6,,
+02/05/2019 07:10:13,Dogsbreath,HIP 20964, 3 B,https://www.dropbox.com/s/eksrrzu8f989yho/Screenshot_0001.bmp?dl=0,Large upright leviathans,1,,,,,,"didn't have a sensor but 1 door looked accessible and I could see beyond, the other two looked blocked",4,,1,,
+02/05/2019 07:26:31,Armourer,TAURUS DARK REGION MY-R B4-1, 1 B,http://www.image-share.com/ijpg-3955-168.html,Large upright leviathans,4,,,,,,,5,,5,,
+02/05/2019 17:23:25,G_Bison,PLEIADES SECTOR DG-X C1-11, A 3 A,https://i.imgur.com/1q2uqIH.jpg,Small Reposing Leviathans,0,N/A,No,,,,Inactive Site,5,"All Blocked, Inactive Site",1,,
+02/05/2019 23:10:58,LCU No Fool Like One,TAURUS DARK REGION JH-V C2-9, AB 1 A,https://i.imgur.com/K2PWBUB.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,2,,4,,
+02/05/2019 23:41:43,LCU No Fool Like One,HIP 22334, 1 D,https://i.imgur.com/SLK3PCN.jpg,"Large upright leviathans, Small Reposing Leviathans",,Yes to Central Chamber,Yes,,,,,2,,5,,
+03/05/2019 03:50:20,ElectricNacho,DELPHI, 5 A,https://drive.google.com/file/d/1BrDq9RmgGqn_BrmQQ5jcdVUPUKl9-KHq/view?usp=sharing,Small Reposing Leviathans,,,,,,,,5,,4,,
+03/05/2019 22:58:01,LCU No Fool Like One,HIP 21348, A 2 B,https://i.imgur.com/2cdB4xJ.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,4,,
+03/05/2019 23:13:50,LCU No Fool Like One,HIP 20859, A 1 A,https://i.imgur.com/tMoZIDN.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,4,,
+03/05/2019 23:38:42,LCU No Fool Like One,PLEIADES SECTOR EL-Y D23, ABCD 1 A,https://i.imgur.com/pv9MA4z.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,5,,
+04/05/2019 00:09:15,LCU No Fool Like One,TAURUS DARK REGION OX-U C2-10, 4 B,https://i.imgur.com/hD5XqtV.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,4,,
+04/05/2019 08:07:29,LCU No Fool Like One,SYNUEFE HZ-M D8-69, B 7 F,https://i.imgur.com/xy0gUq5.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,4,,
+04/05/2019 08:34:22,LCU No Fool Like One,HIP 24007, 1 B,https://i.imgur.com/zQIxKJs.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,3,,4,,
+04/05/2019 18:14:44,LCU No Fool Like One,HIP 25274,8 A,https://i.imgur.com/dCxERJT.jpg,Small Reposing Leviathans,,,,,,,,1,,1,,
+04/05/2019 18:29:07,LCU No Fool Like One,COL 285 SECTOR IL-X C1-4,A 2 C,https://i.imgur.com/2JHfAda.jpg,Small Reposing Leviathans,,,,,,,,2,,2,,
+04/05/2019 18:41:46,LCU No Fool Like One,COL 285 SECTOR IL-X C1-16,A 4 A,https://i.imgur.com/4Yx8feo.jpg,No Leviathans,,,,,,,,5,,0,,
+04/05/2019 20:08:04,LCU No Fool Like One,HIP 22851,7 A,https://i.imgur.com/xqoRXWa.jpg,Small Reposing Leviathans,,,,,,,,1,,1,,
+04/05/2019 20:19:01,LCU No Fool Like One,SYNUEFE UZ-X C17-10,1 B,https://i.imgur.com/8RhMhTO.jpg,Small Reposing Leviathans,,,,,,,Quite a few bones in the recesses,4,,1,,
+04/05/2019 20:30:55,LCU No Fool Like One,SYNUEFE UZ-X C17-12,AB 2 A,https://i.imgur.com/hqGXCoO.jpg,No Leviathans,,,,,,,,4,,0,,
+04/05/2019 20:42:30,LCU No Fool Like One,SYNUEFE WU-X C17-8,2 A,https://i.imgur.com/U4CKPnq.jpg,Small Reposing Leviathans,,,,,,,,1,,2,,
+04/05/2019 21:03:08,LCU No Fool Like One,SYNUEFE YP-X C17-1,5 C,https://i.imgur.com/vfNqsHv.jpg,"Large upright leviathans, Small Reposing Leviathans",,,Yes,,,,,3,Block on the floor at entrance to central chamber can be bypassed,4,,
+04/05/2019 23:19:12,LCU No Fool Like One,SYNUEFE UK-V B35-0,A 4 A,https://i.imgur.com/WY9OxvB.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,,,4,,
+04/05/2019 23:20:31,LCU No Fool Like One,SYNUEFE UK-V B35-0,A 4 A,https://i.imgur.com/WY9OxvB.jpg,,,,,,,,,1,,4,,
+04/05/2019 23:26:26,LCU No Fool Like One,SYNUEFE YP-X C17-8,2 A,https://i.imgur.com/UPaQPIV.jpg,Small Reposing Leviathans,,,,,,,,5,,1,,
+04/05/2019 23:40:45,LCU No Fool Like One,SYNUEFE CW-V C18-6,A 3 A,https://i.imgur.com/zRsgYaa.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,3,,4,,
+05/05/2019 00:04:10,LCU No Fool Like One,SYNUEFE OV-K D9-56,9 C,https://i.imgur.com/M2mVc8W.jpg,"Large upright leviathans, Small Reposing Leviathans",3,,Yes,,,,,5,,4,,
+05/05/2019 14:53:41,Delmonte,HIP 18730,3 G A,https://cdn.discordapp.com/attachments/296717350575800320/574593902456209433/HIP_18730HIP_18730_3_g_a_00005.png,Small Reposing Leviathans,3,Yes,Yes,,,,,2,One blocked door,4,,
+05/05/2019 16:08:00,Delmonte,PLEIADES SECTOR MM-V B2-2,1 B,https://cdn.discordapp.com/attachments/296717350575800320/574612876153847818/Pleiades_Sector_MM-V_b2-2Pleiades_Sector_MM-V_b2-2_1_b_00001.png,,4,Yes,Yes,,,,"There once was a Thargoid Maid called Evie, Who milked the scavengers twice weekly..........",2,No,5,,
+05/05/2019 16:44:42,Delmonte,HIP 19549,A 2 C,https://cdn.discordapp.com/attachments/296717350575800320/574620251300560906/HIP_19549HIP_19549_A_2_c_00002.png,Small Reposing Leviathans,3,Yes,Yes,,,,One day inevitability she grabbed the appendage of Thargoid gentry ....,3,Yes one door,4,,
+05/05/2019 17:03:42,Henoch,PLEIADES SECTOR MM-V B2-2,1 B,https://imgur.com/AHGqXtM,Large upright leviathans,,,,,,,,1,,5,,
+05/05/2019 17:05:52,Henoch,PLEIADES SECTOR MM-V B2-2,1B,https://imgur.com/ZWGZSKM,Large upright leviathans,,,No,,,,,1,,5,,
+05/05/2019 17:07:14,Henoch,PLEIADES SECTOR MM-V B2-2,1 B,https://imgur.com/JXLvqmz,Large upright leviathans,,,No,,,,,1,,5,,
+05/05/2019 17:12:54,Delmonte,HIP 21251,A 8 C,https://cdn.discordapp.com/attachments/296717350575800320/574627797939650580/HIP_21251HIP_21251_A_8_c_00001.png,,4,To the Map room Yes,Yes,,,,"He pushed her blushes away, and now she milks him thrice daily :-) ",5,None,4,,
+05/05/2019 17:46:18,Delmonte,TAURUS DARK REGION IR-W D1-47,A 3 A,https://cdn.discordapp.com/attachments/296717350575800320/574633970914820096/Taurus_Dark_Region_IR-W_d1-47Taurus_Dark_Region_IR-W_d1-47_A_3_a_00001.png,,4,,Yes,,,,Exits stage left ;-) ,5,None,3,,
+05/05/2019 18:05:03,G_Bison,HIP 20486,A 3 A,https://i.imgur.com/a1HDK5a.jpg,Large upright leviathans,4,N/A,Yes,,,,,1,None from visusal ,4,,
+05/05/2019 19:19:36,G_Bison,SYNUEFE RT-Z C16-13,1 A,https://i.imgur.com/NSx4OXy.jpg,Large upright leviathans,4,N/A,,,,,,1,N/A,4,,
+05/05/2019 23:23:14,LCU No Fool Like One,ARIES DARK REGION JW-W C1-4,7 A,https://i.imgur.com/6X4Ld4d.jpg,,,,,,,,,1,,2,,
+05/05/2019 23:34:34,LCU No Fool Like One,HIP 18368,2 H,https://i.imgur.com/TANhbFy.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,5,,6,,
+05/05/2019 23:46:03,LCU No Fool Like One,PLEIADES SECTOR MC-V C2-8,8 B,https://i.imgur.com/9WkbzCl.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,4,,
+06/05/2019 00:03:30,LCU No Fool Like One,PLEIADES SECTOR MC-V C2-6,B 4 A,https://i.imgur.com/fYMnFwT.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,2,,4,,
+06/05/2019 08:32:50,Kace Mono,HIP 22347,4 A,https://i.imgur.com/e7zwBhy.jpg,Large upright leviathans,,,,,,,,5,,0,,
+07/05/2019 05:35:16,G_Bison,HIP 20830,A 8 F,https://i.imgur.com/4VdR0iJ.jpg,Large upright leviathans,4,N/A,Yes,,,,Thargoid Links were detectable while on approach in ship.,5,N/A,4,,
+08/05/2019 19:03:33,Armourer,HIP 14756,2 E,http://www.image-share.com/upload/3957/184.jpg,Large upright leviathans,3,,,,,,,2,,2,,
+08/05/2019 19:26:24,Armourer,COL 285 SECTOR EB-X D1-39,4 A,http://www.image-share.com/upload/3957/186.jpg,Small Reposing Leviathans,3,,,,,,,1,,1,,
+11/05/2019 03:45:32,MrMerdur,HYADES SECTOR DB-X C1-8,3 A,,,,,,,,,this is first thargoid site I've visited. I didn't know what to look for and couldn't get inside.,2,,1,,
+12/05/2019 21:41:13,Mazaious,ARIES DARK REGION GW-W D1-86,1 B,https://i.imgur.com/oPCW2Bu.jpg,No Leviathans,,,,,,,,4,,0,,
+12/05/2019 22:10:17,Mazaious,HYADES SECTOR DB-X C1-19,8 C,https://i.imgur.com/cGOhtjA.jpg,,,,,,,,,4,,0,,
+13/05/2019 00:58:15,Hrdina,SYNUEFAI RK-Y C17-3,A 1 A,https://i.imgur.com/xnL1HGr.png,,,,,,,,"I didn't go inside or anything, and haven't spent much time with Thargoid sites, so sorry I didn't have answers to any of the extra questions. I did see a bunch of Scavengers and some Barnacle Barbs.",,,4,,
+17/05/2019 00:36:00,LCU No Fool Like One,ARIES DARK REGION FW-W D1-59,A 5 A,https://i.imgur.com/VyIYuNl.jpg,Small Reposing Leviathans,,,,,,,,2,,2,,
+19/05/2019 19:24:33,LCU No Fool Like One,ARIES DARK REGION MX-U C2-10,1 C,https://i.imgur.com/GKFZN5w.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,4,,
+19/05/2019 19:36:20,LCU No Fool Like One,ARIES DARK REGION JC-V C2-6,A 4 A,https://i.imgur.com/ZtkKZOd.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,5,,4,,
+19/05/2019 19:55:51,LCU No Fool Like One,HIP 14702,1 C,https://i.imgur.com/HvHPtm7.jpg,Small Reposing Leviathans,,,,,,,,5,,1,,
+19/05/2019 20:14:42,LCU No Fool Like One,HIP 15443,AB 4 B,https://i.imgur.com/l4vjEzG.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,2,,4,,
+19/05/2019 20:25:23,LCU No Fool Like One,HIP 14746,6 A,https://i.imgur.com/3ElGv8p.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,2,,4,,
+19/05/2019 20:42:32,LCU No Fool Like One,SYNUEFE MK-M D8-20,A 2 A,https://i.imgur.com/wqG9hBe.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,4,,
+19/05/2019 20:53:30,LCU No Fool Like One,MEL 22 SECTOR DG-O C6-0,2 A,https://i.imgur.com/tRfbGzO.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,3,,4,,
+19/05/2019 22:18:00,LCU No Fool Like One,MEL 22 SECTOR BV-P C5-2,1 A,https://i.imgur.com/krPqfV9.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,5,,
+19/05/2019 22:30:06,LCU No Fool Like One,MEL 22 SECTOR UE-G B11-0,A 2,https://i.imgur.com/c5Fdi11.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,3,,4,,
+19/05/2019 22:44:24,LCU No Fool Like One,HIP 18117,5 D,https://i.imgur.com/wco9dOe.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,4,,4,,
+19/05/2019 23:01:39,LCU No Fool Like One,HIP 18778,A 2 A,https://i.imgur.com/5nwiSn8.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,4,,
+19/05/2019 23:13:33,LCU No Fool Like One,HIP 20318,3 A,https://i.imgur.com/ScaN4SX.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,5,,4,,
+19/05/2019 23:24:05,LCU No Fool Like One,STRUVE'S LOST SECTOR NN-T C3-6,1 C,https://i.imgur.com/OEAFl39.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,4,,
+19/05/2019 23:45:11,LCU No Fool Like One,STRUVE'S LOST SECTOR NN-T C3-1,ABCD 1 A,https://i.imgur.com/8dU4SCs.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,5,,
+20/05/2019 00:07:26,LCU No Fool Like One,MEL 22 SECTOR MC-V D2-19,BC 1 B,https://i.imgur.com/Tqk8eVk.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,5,,
+20/05/2019 00:25:06,LCU No Fool Like One,PLEIADES SECTOR KM-W D1-40,B 10 A,https://i.imgur.com/l2sxD0B.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,4,,
+20/05/2019 00:49:11,LCU No Fool Like One,SYNUEFAI UZ-M D8-63,B 1 A,https://i.imgur.com/NAxpizb.jpg,"Large upright leviathans, Small Reposing Leviathans",,,,,,,,1,,4,,
+21/05/2019 12:15:15,Denholm,COL 285 SECTOR BQ-X C1-10,1 A,https://cdn.discordapp.com/attachments/180430318610808832/580313500384231445/SPOILER_unknown.png,,,,,,,,,3,,2,,
+23/05/2019 12:35:39,MadDavo,HYADES SECTOR DB-X C1-19,8 C,https://i.imgur.com/62b7MM4.png,,,,,,,,,3,,0,,
+24/05/2019 01:16:49,Crunch Buttsteak,HIP 22711,7 A,https://i.imgur.com/Xx0wnpo.jpg,Small Reposing Leviathans,,,,,,,,3,,3,,
+24/05/2019 07:27:30,MadDavo,MEL 22 SECTOR JC-V D2-15,ABC 1 A,https://i.imgur.com/R0bYMbG.png,,,,,,,,,5,,6,,
+26/05/2019 01:30:21,ElectricNacho,HYADES SECTOR DB-X C1-19,8 C,https://drive.google.com/file/d/11fE2j2AqjOdwYsyz4pXcQIf2FIBtrpFy/view?usp=sharing,No Leviathans,,,,,,,,5,,0,,
+27/05/2019 08:02:36,Crunch Buttsteak,PLEIADES SECTOR JM-W D1-52,2 A,https://i.imgur.com/EF8RPWd.png,Small Reposing Leviathans,3,No,Yes,,,,Forgot to get a TP before entering site. Apologies for no results. ,3,One exterior door blocked,4,,
+16/06/2019 15:00:56,LordTyvin,HYADES SECTOR DB-X C1-19,8 C,https://i.imgur.com/RT1Ma5f.jpg,,,,,,,,,4,,0,,
+06/07/2019 02:48:59,ElectricNacho,HYADES SECTOR DB-X C1-19,8 C,https://photos.google.com/share/AF1QipMu1f-PYbe2otSa1Ay_7IJFlDVcv-F0bxsPFm9jfg0lMvRedLaidlLLagHSj4wFnA/photo/AF1QipO_DHaorehP9rDbqdVqwG_nLZQg3wU-ZKwnhVxL?key=VXp0M0R1b2xQUnZYa3RPVi1hWVpBUFZjVGtjSGJn,No Leviathans,,,,,,,,5,,0,,
+06/07/2019 03:02:43,ElectricNacho,HYADES SECTOR DB-X C1-8,3 A,https://i.imgur.com/xQYrNC7.jpg,Small Reposing Leviathans,,,,,,,,4,,1,,
+07/07/2019 09:44:59,MadDavo,HYADES SECTOR DB-X C1-8,3 A,https://i.imgur.com/ja0y8i0.png,Small Reposing Leviathans,,,,,,,,3,,1,,
+01/09/2019 01:21:50,ByteSturm,HIP 16704,5 A,https://i.imgur.com/1rdRSYV.jpg,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Pleiades Sector LN-T c3-4 https://tool.canonn.tech/linkdecoder/?origin=HIP+16704&data=hlh+lhl+%3B+hll+lll+hlh%0D%0Ahhl+hhh+hll+%3B+lll+llh+lhh%0D%0Ahlh+hhh+lhl+lhl+%3B+hhl+lll+lhl+hhh,"Lusonda
+https://tool.canonn.tech/linkdecoder/?origin=HIP+16704&data=lll+hhl+%3B+hhl+lll+lhl%0D%0Ahhl+lhl+%3B+hll+hhl%0D%0Alhh+llh+lhl+%3B+hll+lll+hlh
+",,It has 1 cave-in in the tunnels and 1 cave-in by a(n exterior) door.,4,Yes.,4,,
+01/09/2019 01:25:07,ByteSturm,Mel 22 Sector JC-V d2-14,A 4 A,https://i.imgur.com/TtzcyIx.png,,4,"Yes, 4 around the central chamber.",Yes,Pleiades Sector IH-V c2-16 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+JC-V+d2-14&data=hlh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+hhl+hll+%3B+hll+hhl+hhh,"Ngaiawang
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+JC-V+d2-14&data=hhl+hlh+hll+%3B+hll+hhl+hhh%0D%0Ahhl+%3B+hlh%0D%0Ahlh+hhl+hlh+lhl+%3B+hhl+lll+lhl+hhh
+",,"I drove one eve,
+Long hallowed halls,
+Saw an alien,
+And realized I had no balls.",1,No.,6,,
+01/09/2019 01:32:58,ByteSturm,Mel 22 Sector JC-V d2-15,ABC 1 A,https://i.imgur.com/WCsYbBA.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Mel 22 Sector IO-G b11-1 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+JC-V+d2-15&data=hlh+hll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+%3B+hhl+lhh+lhh%0D%0Ahhl+hhh+lhh+hll+%3B+lll+llh+lhh,"Mel 22 Sector JC-V d2-41
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+JC-V+d2-15&data=lhh+lhl+%3B+hll+lll+hlh%0D%0Ahll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhl+hhl+%3B+hhl+lll+lhl
+","Mel 22 Sector JC-V d2-14
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+JC-V+d2-15&data=hhl+lll+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhh+llh+lhl+%3B+hhl+lll+lhl+hhh
+","Got a Thargoid visitor after activating the structure and exiting it. But the damn thing glitched out and kept bouncing off the ground up a nearby mountain. I still had some stuff to do in the structure so I think it just ended up making it over the mountain and flying away.
+",5,No.,6,,
+01/09/2019 01:39:53,ByteSturm,Mel 22 Sector OY-R c4-5,2 A,https://i.imgur.com/gEwvMGJ.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Mel 22 Sector JC-V d2-14 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+OY-R+c4-5&data=hhl+lll+lll+%3B+hhl+lll+lhl+hhh%0D%0Alhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhh+llh+lhl+%3B+hhl+lll+lhl+hhh,"Mel 22 Sector JC-V d2-15
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+OY-R+c4-5&data=lhh+hhl+%3B+hll+lll+hlh%0D%0Ahll+lll+%3B+hhl+lll+lhl+hhh%0D%0Alhh+hhl+lhl+%3B+hll+lll+hlh
+","Mel 22 Sector IO-G B11-1
+LINK POINTS IN SYSTEM
+","Another site down,
+Another off the list.
+Scores more to go,
+I'm starting to feel pissed.",2,No.,5,,
+01/09/2019 01:41:54,ByteSturm,Mel 22 Sector IO-G b11-1,1 A,https://i.imgur.com/bNwtnhK.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Pleiades Sector JC-U b3-2 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+IO-G+b11-1&data=lll+%3B+hll+lll+hlh%0D%0Alhl+hhl+%3B+hll+lll+hlh%0D%0Ahhl+lhh+hll+%3B+hhl+lhh+lhh,"Brib
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+IO-G+b11-1&data=lll+hhh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhh+lll+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhl+hhl+lll+%3B+hhl+lll+lhl+hhh
+",,"Hippity hoppity,
+The aliens wants me off their property.",5,No.,4,,
+01/09/2019 01:50:57,ByteSturm,Mel 22 Sector JC-V d2-31,A 16 B,https://i.imgur.com/T7XtZ5F.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Pleiades Sector JN-S b4-3 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+JC-V+d2-31&data=lll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hlh+hll+%3B+lll+llh+lhh%0D%0Ahlh+lhl+%3B+hlh+lhh,"Thraskias
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+JC-V+d2-31&data=lll+hll+%3B+hhl+lll+lhl%0D%0Ahll+%3B+lhl%0D%0Ahlh+hlh+hll+lll+%3B+hhl+lll+lhl+hhh
+",,"Some miles driven,
+Some fuel spent,
+THE EMPEROR APPROVES,
+AND I SHALL MAKE THE XENOS REPENT!",2,No.,6,,
+01/09/2019 01:52:28,ByteSturm,Mel 22 Sector HH-V d2-37,AB 1 A,https://i.imgur.com/gMJAsPT.jpg,,4,"Yes, 4 around the central chamber.",Yes,G 69-11 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+HH-V+d2-37&data=llh+hhh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lhh+hhl+%3B+hll+hhl+hhh%0D%0Ahhl+hhl+hll+hhl+%3B+lll+llh+lhh,"HIP 17225
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+HH-V+d2-37&data=lll+%3B+hll+lll+hlh%0D%0Ahhl+%3B+hhl+hlh%0D%0Ahll+lll+hhl+%3B+hll+lll+hlh
+",,"Monotony sets in,
+My SRV feels inert.
+I scan another chamber
+As a Goid egg goes 'splurt'.",1,No.,3,,
+01/09/2019 01:54:48,ByteSturm,Mel 22 Sector HH-V d2-53,1 B,https://i.imgur.com/X9wAekp.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Taurus Dark Region CL-Y d53 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+HH-V+d2-53&data=hlh+hhl+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhl+%3B+hll+lll+hlh%0D%0Ahlh+hhh+hhl+lhl+%3B+hhl+lll+lhl+hhh,"Mel 22 Sector JX-T c3-9
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+HH-V+d2-53&data=lhh+%3B+hll+hhl%0D%0Alll+%3B+hll+hhl+hhh%0D%0Ahlh+hhh+llh+%3B+hhl+lll+lhl
+","Taurus Dark Region MY-R b4-1
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+HH-V+d2-53&data=hhl+hhh+lll+%3B+lll+llh+lhh%0D%0Ahlh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhh+hlh+hhl+%3B+lll+llh+lhh
+","Got another Thargoid visitor, but it had left by the time I reached my ship. It didn't even make it close to the site, it was there for all of about 10 seconds.
+
+It has 1 cave-in by a(n exterior) door.
+",2,Yes.,3,,
+01/09/2019 01:56:09,ByteSturm,Mel 22 Sector FH-U c3-9,A 1 A,https://i.imgur.com/j2QmGRV.jpg,,4,"Yes, 4 around the central chamber.",Yes,HIP 23022 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+FH-U+c3-9&data=lll+lll+%3B+lll+llh+lhh%0D%0Ahhl+lhl+hll+%3B+lll+llh+lhh%0D%0Ahll+llh+lll+%3B+hll+lll+hlh,"Oitbi
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+FH-U+c3-9&data=hhl+%3B+hlh%0D%0Ahhl+hhl+llh+lll+%3B+hhl+lll+lhl+hhh%0D%0Allh+hll+%3B+lhl+hhh
+",,"I've started a race
+To get through this place
+Before a random Goid
+Scatters my ship to the void.",1,No.,6,,
+01/09/2019 02:01:53,ByteSturm,Taurus Dark Region MY-R b4-1,1 B,https://i.imgur.com/7wff0ia.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Taurus Dark Region CL-Y d53 https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+MY-R+b4-1&data=hlh+hhl+lhl+%3B+hhl+lll+lhl+hhh%0D%0Alll+%3B+hll+hhl+hhh%0D%0Ahlh+hhh+hhl+lhl+%3B+hhl+lll+lhl+hhh,"Mel 22 Sector FH-U c3-9
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+MY-R+b4-1&data=hlh+hhl+%3B+hhl+lhh+lhh%0D%0Ahll+%3B+hhl+lhh+lhh%0D%0Ahlh+hhh+lhl+lhl+%3B+hhl+lll+lhl+hhh
+","Mel 22 Sector HH-V d2-53
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+MY-R+b4-1&data=hlh+hlh+%3B+hhl+lll+lhl%0D%0Ahlh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Alhh+hhl+hll+%3B+hll+lll+hlh
+","My third Thargoid visitor at an active site, but this one was also bugged in some fashion. It floated in position almost a kilometer away from the site doing nothing.
+",4,No.,5,,
+01/09/2019 02:26:09,ByteSturm,Mel 22 Sector DB-X d1-46,A 8 A,https://i.imgur.com/TG15nG9.jpg,,4,"Yes, 4 around the central chamber.",Yes,Deciat https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+DB-X+d1-46&data=llh+lll+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+lhl+%3B+hll+hhl+hhh%0D%0Ahlh+hhh+llh+%3B+hhl+lll+lhl,"Deciat
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+DB-X+d1-46&data=llh+lll+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+lhl+%3B+hll+hhl+hhh%0D%0Ahlh+hhh+llh+%3B+hhl+lll+lhl
+",,"I've come across
+A geological aberration,
+The center of which
+Hides a bright constellation.",1,No.,4,,
+01/09/2019 02:29:19,ByteSturm,Mel 22 Sector JX-T c3-9,6 A,https://i.imgur.com/tShCKvz.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,HIP 7158 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+JX-T+c3-9&data=lhl+lhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahll+lll+hll+%3B+lll+llh+lhh%0D%0Ahlh+hhl+lhl+%3B+hhl+lll+lhl,"Dulerce
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+JX-T+c3-9&data=hhl+hhl+lll+%3B+hll+hhl+hhh%0D%0Ahlh+hhh+lhl+%3B+hll+lll+hlh%0D%0Ahlh+hhl+hll+lhl+%3B+hhl+lll+lhl+hhh
+",,"Flitting red eyes
+Flit through the night,
+Droning 'long
+The halls of this site.",3,No.,5,,
+01/09/2019 02:33:10,ByteSturm,HIP 15889,11 B,https://i.imgur.com/7ULeu7X.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Mel 22 Sector JX-T c3-9 https://tool.canonn.tech/linkdecoder/?origin=HIP+15889&data=lhh+%3B+hll+hhl%0D%0Alll+%3B+hll+lll+hlh%0D%0Ahlh+hhh+llh+%3B+hhl+lll+lhl,"Mel 22 Sector HH-V d2-37
+https://tool.canonn.tech/linkdecoder/?origin=HIP+15889&data=lll+lhl+%3B+lll+llh+lhh%0D%0Alll+%3B+hll+lll+hlh%0D%0Ahll+hlh+hhl+%3B+hll+hhl+hhh
+","HIP 16704
+https://tool.canonn.tech/linkdecoder/?origin=HIP+15889&data=hll+%3B+lhl+hhh%0D%0Ahhl+hhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+hhl+hll+%3B+hll+hhl+hhh
+","I've come across
+A geological aberration,
+The center of which
+Hides a bright constellation.",5,No.,4,,
+01/09/2019 02:35:32,ByteSturm,HIP 16795,A 10 F,https://i.imgur.com/p9srY4g.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Taurus Dark Region CL-Y d53 https://tool.canonn.tech/linkdecoder/?origin=HIP+16795&data=hlh+hhl+lhl+%3B+hhl+lll+lhl+hhh%0D%0Alll+%3B+hll+lll+hlh%0D%0Ahlh+hhh+hhl+lhl+%3B+hhl+lll+lhl+hhh,"HIP 15889
+https://tool.canonn.tech/linkdecoder/?origin=HIP+16795&data=hlh+hlh+%3B+hhl+lll+lhl%0D%0Alll+%3B+hll+lll+hlh%0D%0Ahhl+hhh+hlh+hhl+%3B+lll+llh+lhh
+","Mel 22 Sector DB-X d1-46
+https://tool.canonn.tech/linkdecoder/?origin=HIP+16795&data=hll+%3B+hlh+lhh%0D%0Ahhl+lhl+%3B+lll+llh+lhh%0D%0Ahlh+hhh+lhh+%3B+hhl+lll+lhl
+","Flying through the dark
+The sight before me is stark.
+Spires litter the ground
+Of the next site I have found.",5,No.,3,,
+01/09/2019 02:37:06,ByteSturm,HIP 15889,11 B,https://i.imgur.com/7ULeu7X.jpg,,4,"Yes, 4 around the central chamber.",Yes,Mel 22 Sector JX-T c3-9 https://tool.canonn.tech/linkdecoder/?origin=HIP+15889&data=lhh+%3B+hll+hhl%0D%0Alll+%3B+hll+lll+hlh%0D%0Ahlh+hhh+llh+%3B+hhl+lll+lhl,"Mel 22 Sector HH-V d2-37
+https://tool.canonn.tech/linkdecoder/?origin=HIP+15889&data=lll+lhl+%3B+lll+llh+lhh%0D%0Alll+%3B+hll+lll+hlh%0D%0Ahll+hlh+hhl+%3B+hll+hhl+hhh
+","HIP 16704
+https://tool.canonn.tech/linkdecoder/?origin=HIP+15889&data=hll+%3B+lhl+hhh%0D%0Ahhl+hhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+hhl+hll+%3B+hll+hhl+hhh
+","I've come across
+A geological aberration,
+The center of which
+Hides a bright constellation.",1,No.,4,,
+11/09/2019 00:08:13,ByteSturm,Taurus Dark Region CL-Y d53,A 4,https://i.imgur.com/mLuSZqD.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, though unclear how many and where.",Yes,Ceti Sector DL-X b1-6 (?) https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+CL-Y+d53&data=lhl+lhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lhh+hll+%3B+hll+hhl+hhh%0D%0Ahlh+hhl+lhl+%3B+hhl+lll+lhl,"HIP 13806(?)
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+CL-Y+d53&data=lll+hll+%3B+hll+hhl+hhh%0D%0Allh+hll+%3B+hhl+lll+hlh%0D%0Ahlh+hhl+llh+%3B+hhl+lll+lhl
+",,"Some miles driven,
+Some fuel spent,
+THE EMPEROR APPROVES,
+AND I SHALL MAKE THE XENOS REPENT!",2,Unknown.,3,,
+11/09/2019 00:11:28,ByteSturm,Taurus Dark Region FW-W c1-3,1 A,https://i.imgur.com/6eUTiU5.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, although it is unclear where and how many.",Yes,Result: The three spheres do not intersect! https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+FW-W+c1-3&data=hlh+hhh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+lll+%3B+lll+llh+lhh%0D%0Ahll+llh+hll+%3B+hll+lll+hlh,"Taurus Dark Region OJ-Q b5-3
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+FW-W+c1-3&data=hhl+hhh+lll+%3B+lll+llh+lhh%0D%0Ahhl+hll+%3B+hll+lll+hlh%0D%0Ahhl+hhh+hlh+hhl+%3B+lll+llh+lhh
+","Mel 22 Sector IS-S c4-6
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+FW-W+c1-3&data=hhl+hlh+hll+%3B+lll+llh+lhh%0D%0Ahhl+lhh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhh+lhl+lhl+%3B+hhl+lll+lhl+hhh
+","Hippity hoppity,
+The aliens wants me off their property.",3,Unknown.,5,,
+11/09/2019 00:17:45,ByteSturm,Taurus Dark Region BQ-Y c12,2 A,https://i.imgur.com/a3T7bVp.jpg,,4,"Yes, although unclear where and how many.",Yes,HIP 21373 https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+BQ-Y+c12&data=hlh+hll+%3B+hhl+lll+lhl%0D%0Allh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lll+hhl+hhl+%3B+hhl+lll+lhl+hhh,"HIP 20989
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+BQ-Y+c12&data=hhl+hhl+lhl+%3B+lll+llh+lhh%0D%0Alhh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+llh+hhl+%3B+hll+lll+hlh
+","Taurus Dark Region DL-Y d54
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+BQ-Y+c12&data=hlh+hhh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Alll+%3B+hll+hhl+hhh%0D%0Ahll+llh+hll+%3B+hll+lll+hlh
+","Another site down,
+Another off the list.
+Scores more to go,
+I'm starting to feel pissed.",1,Unknown.,5,,
+11/09/2019 00:22:18,ByteSturm,HIP 19665,8 B,https://i.imgur.com/pXz5fq0.jpg,,4,"Yes, unclear how many and where.",Yes,Pleiades Sector DQ-Y c4 https://tool.canonn.tech/linkdecoder/?origin=HIP+19665&data=hhl+lhh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+lll+%3B+lll+llh+lhh%0D%0Alll+hlh+lhl+%3B+lll+llh+lhh,"HIP 20644
+https://tool.canonn.tech/linkdecoder/?origin=HIP+19665&data=hlh+hlh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+lhl+%3B+lll+llh+lhh%0D%0Ahhl+llh+hll+hll+%3B+hhl+lll+lhl+hhh
+","Taurus Dark Region QN-T b3-0
+https://tool.canonn.tech/linkdecoder/?origin=HIP+19665&data=hll+lhl+%3B+hll+hhl+hhh%0D%0Ahhl+%3B+hll+hhl%0D%0Ahhl+llh+hlh+hhl+%3B+hhl+lll+lhl+hhh
+","Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.",1,Unknown.,3,,
+11/09/2019 00:23:38,ByteSturm,Pleiades Sector DQ-Y c4,6 A,https://i.imgur.com/xRafl0H.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, unknown where and how many.",Yes,HIP 19792 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+DQ-Y+c4&data=lll+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lll+lhh+lll+%3B+hhl+lll+lhl+hhh,"Dhanhopi
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+DQ-Y+c4&data=lll+hhh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lhl+lhl+%3B+hll+hhl+hhh%0D%0Ahhl+hhl+lhh+hhl+%3B+lll+llh+lhh
+",,"Monotony sets in,
+My SRV feels inert.
+I scan another chamber
+As a Goid egg goes 'splurt'.",5,Unknown.,5,,
+11/09/2019 00:26:20,ByteSturm,Pleiades Sector GG-Y d33,3 A,https://i.imgur.com/ZhyY5kb.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, unclear how many and where.",Yes,Pleiades Sector LY-Q b5-2 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+GG-Y+d33&data=hhl+lhh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hll+%3B+hhl+lhh+lhh%0D%0Ahlh+hhh+llh+lll+%3B+hhl+lll+lhl+hhh,"Kung Mu
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+GG-Y+d33&data=hlh+lll+lll+%3B+lll+llh+lhh%0D%0Alll+hhl+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+lll+%3B+hll+hhl
+",,"I've started a race
+To get through this place
+Before a random Goid
+Scatters my ship to the void.",5,Unknown.,6,,
+11/09/2019 00:27:56,ByteSturm,Struve's Lost Sector OI-T c3-3,2 B,https://i.imgur.com/AAMY5cJ.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, unclear how many or where.",Yes,Pleiades Sector DQ-Y c4 https://tool.canonn.tech/linkdecoder/?origin=Struve%27s+Lost+Sector+OI-T+c3-3&data=hhl+lhh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Alll+%3B+hhl+lll+lhl+%0D%0Alll+hlh+lhl+%3B+lll+llh+lhh,"HIP 18117
+https://tool.canonn.tech/linkdecoder/?origin=Struve%27s+Lost+Sector+OI-T+c3-3&data=hhl+hhl+lhl+%3B+lll+llh+lhh%0D%0Alhh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+hll+lhl+%3B+hll+lll+hlh
+","Pleiades Sector GG-Y d33
+https://tool.canonn.tech/linkdecoder/?origin=Struve%27s+Lost+Sector+OI-T+c3-3&data=lhl+lll+%3B+lll+llh+lhh%0D%0Ahll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+hll+hll+%3B+hhl+lll+lhl+hhh
+","I've come across
+A geological aberration,
+The center of which
+Hides a bright constellation.",5,Unknown.,4,,
+11/09/2019 00:31:06,ByteSturm,HIP 16985,A 4 E,https://i.imgur.com/tfA6n2R.jpg,,4,"Yes, unclear how many or where.",Yes,Result: The three spheres do not intersect! https://tool.canonn.tech/linkdecoder/?origin=HIP+16985&data=hlh+hlh+%3B+hhl+lll+lhl%0D%0Alhh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+llh+lhl+%3B+hhl+lll+lhl+hhh,"Mel 22 Sector BV-P c5-2
+https://tool.canonn.tech/linkdecoder/?origin=HIP+16985&data=hlh+lhh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Alhh+lhl+%3B+lll+llh+lhh%0D%0Ahlh+llh+hll+%3B+hll+hhl+hhh
+","Mel 22 Sector UE-G b11-0
+https://tool.canonn.tech/linkdecoder/?origin=HIP+16985&data=lll+lhl+%3B+lll+llh+lhh%0D%0Ahll+%3B+hhl+lhh+lhh%0D%0Ahhl+llh+hll+hhl+%3B+hhl+lll+lhl+hhh
+","Flitting red eyes
+Flit through the night,
+Droning 'long
+The halls of this site.",1,Unknown.,6,,
+11/09/2019 00:34:07,ByteSturm,Mel 22 Sector MX-U d2-14,AB 1 A,https://i.imgur.com/zdxzE9J.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, unclear how many and where.",Yes,HIP 15329 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+MX-U+d2-14&data=lll+hhl+%3B+hll+lll+hlh%0D%0Alhl+llh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+lhh+hll+%3B+hll+hhl+hhh,"Argestes
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+MX-U+d2-14&data=hlh+lll+hll+%3B+lll+llh+lhh%0D%0Ahhl+hhh+hhh+%3B+hhl+lll+lhl%0D%0Ahlh+hhl+lhl+hhl+%3B+hhl+lll+lhl+hhh
+",,"Flying through the dark
+The sight before me is stark.
+Spires litter the ground
+Of the next site I have found.",5,Unknown.,3,,
+11/09/2019 00:36:05,ByteSturm,Mel 22 Sector TT-R c4-2,3 A,https://i.imgur.com/klTcbys.png,,4,"Yes, unclear how many and where.",Yes,LP 389-95 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+TT-R+c4-2&data=lll+hlh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hlh+lll+%3B+hll+lll+hlh%0D%0Alhh+hll+hhl+%3B+hll+lll+hlh,"HR 1257
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+TT-R+c4-2&data=lhh+lll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Alll+hhh+hll+%3B+hhl+lll+lhl+hhh%0D%0Alhh+hlh+hhl+%3B+hll+lll+hlh
+",,"My engine's humming,
+My heartbeat's thrumming,
+The LZ's not clear,
+The Goids draw near!",1,Unknown.,6,,
+11/09/2019 00:37:46,ByteSturm,Mel 22 Sector KC-V d2-28,1 C,https://i.imgur.com/uhSgc5f.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, unclear how many and where.",Yes,HIP 17862 (?) https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+KC-V+d2-28&data=hhl+hll+%3B+lll+llh+lhh%0D%0Alhh+hhl+%3B+hll+lll+hlh%0D%0Ahhl+lll+lhh+hhl+%3B+hhl+lll+lhl+hhh,"Outotz ST-I d9-6
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+KC-V+d2-28&data=hhl+lhh+hll+lll+%3B+lll+llh+lhh%0D%0Ahlh+hll+lhl+%3B+hhl+lhh+lhh%0D%0Ahhl+hhh+lhl+lhl+%3B+lll+llh+lhh
+",,"I drove one eve,
+Long hallowed halls,
+Saw an alien,
+And realized I had no balls.",5,Unknown.,5,,
+11/09/2019 00:39:30,ByteSturm,Mel 22 Sector NX-U d2-31,3 A,https://i.imgur.com/IJqEQxQ.jpg,,4,"Yes, unknown where and how many.",Yes,Outotz ZC-Y c28-2 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+NX-U+d2-31&data=hlh+hhl+hll+lll+%3B+hhl+lll+lhl+hhh%0D%0Alhh+hll+hll+%3B+hll+lll+hlh%0D%0Ahhl+lhh+hlh+lhl+%3B+hhl+lll+lhl+hhh,"Outotz ST-I d9-6
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+NX-U+d2-31&data=hhl+lhh+hll+lll+%3B+lll+llh+lhh%0D%0Ahhl+lhh+hll+lhl+%3B+lll+llh+lhh%0D%0Ahhl+hhh+lhl+lhl+%3B+lll+llh+lhh
+",,"Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.",1,Unknown.,5,,
+11/09/2019 00:41:14,ByteSturm,Mel 22 Sector NX-U d2-27,5 A,https://i.imgur.com/h25iwZD.jpg,,4,No.,Yes,Mel 22 Sector ZU-P c5-1 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+NX-U+d2-27&data=hlh+hll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahll+hhh+%3B+hll+hhl,"Mel 22 Sector YU-F b11-1
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+NX-U+d2-27&data=hhl+hhl+lhl+%3B+lll+llh+lhh%0D%0Ahll+%3B+hhl+lhh+lhh%0D%0Ahhl+llh+hhl+lhl+%3B+hhl+lll+lhl+hhh
+","Mel 22 Sector NX-U d2-31
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+NX-U+d2-27&data=hlh+hlh+%3B+hhl+lll+lhl%0D%0Ahhl+%3B+hhl+lhh+lhh%0D%0Ahlh+lll+lhl+%3B+hll+hhl+hhh
+",It has some cave-ins.,1,"Yes, unknown how many.",4,,
+11/09/2019 00:44:03,ByteSturm,Mel 22 Sector ZU-P c5-1,4 A,https://i.imgur.com/xvNIJ9P.jpg,,4,"Yes, unknown how many and where.",Yes,HIP 14702 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+ZU-P+c5-1&data=hhl+hhl+hhl+%3B+lll+llh+lhh%0D%0Alhh+lhl+%3B+hll+lll+hlh%0D%0Allh+hhl+%3B+llh+hlh,"LHS 2524
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+ZU-P+c5-1&data=hhl+hhh+hhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lll+lll+%3B+hll+hhl+hhh%0D%0Ahlh+hlh+lll+lhl+%3B+hhl+lll+lhl+hhh
+",,"I've started a race
+To get through this place
+Before a random Goid
+Scatters my ship to the void.",1,No.,4,,
+11/09/2019 00:46:27,ByteSturm,HIP 14909,2 A,https://i.imgur.com/hdNxOCy.jpg,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,HIP 16824 https://tool.canonn.tech/linkdecoder/?origin=HIP+14909&data=hll+hhh+%3B+hhl+lll+lhl%0D%0Alll+%3B+hll+hhl%0D%0Alhl+hll+%3B+lhl+hhh,"Atroco
+https://tool.canonn.tech/linkdecoder/?origin=HIP+14909&data=hhl+lhl+lll+%3B+hll+lll+hlh%0D%0Ahll+lll+hhl+%3B+lll+llh+lhh%0D%0Ahlh+hlh+lll+%3B+hhl+lll+lhl",,It has some cave-ins.,5,"Yes, unknown how many and where.",4,,
+11/09/2019 00:48:43,ByteSturm,Mel 22 Sector CB-O c6-4,3 A,https://i.imgur.com/8udZd5e.jpg,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Mel 22 Sector SK-E b12-1 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+CB-O+c6-4&data=lll+lhl+%3B+lll+llh+lhh%0D%0Alll+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+lhl+%3B+hlh+lhh,"Result: The three spheres do not intersect!
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+CB-O+c6-4&data=hlh+hhh+%3B+hhl+lll+lhl%0D%0Allh+hll+%3B+hhl+lll+lhl+hhh%0D%0Alll+lhh+lll+%3B+lll+llh+lhh
+","Pleiades Sector OS-U c2-6
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+CB-O+c6-4&data=hhl+lhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Alhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lll+llh+%3B+hhl+lll+lhl
+",It has some cave-ins.,4,"Yes, unknown where and how many.",4,,
+11/09/2019 00:50:04,ByteSturm,Pleiades Sector OS-U c2-6,A 5 B,https://i.imgur.com/Ss1zRzK.png,,4,"Yes, unclear where and how many.",Yes,Pleiades Sector KM-W d1-40 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+OS-U+c2-6&data=hhl+hhl+%3B+hhl+lhh+lhh%0D%0Ahhl+hll+%3B+hhl+lll+lhl%0D%0Ahlh+lll+lhl+%3B+hll+hhl+hhh,"HIP 14909
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+OS-U+c2-6&data=hlh+hhh+%3B+hhl+lll+lhl%0D%0Alhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Alll+lhh+lll+%3B+lll+llh+lhh
+","Mel 22 Sector CB-O c6-4
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+OS-U+c2-6&data=hll+lhl+%3B+hll+hhl+hhh%0D%0Alhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lll+lll+lll+%3B+hhl+lll+lhl+hhh
+","I've come across
+A geological aberration,
+The center of which
+Hides a bright constellation.",1,No.,4,,
+11/09/2019 00:55:17,ByteSturm,Pleiades Sector JM-W d1-52,2 A,https://i.imgur.com/68QYPWx.jpg,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Pleiades Sector OS-U c2-6 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+JM-W+d1-52&data=hhl+lhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Allh+%3B+hhl+lll+lhl%0D%0Ahhl+lll+llh+%3B+hhl+lll+lhl,"Pleiades Sector OS-U c2-7
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+JM-W+d1-52&data=lhl+lll+%3B+lll+llh+lhh%0D%0Ahlh+lll+%3B+lll+llh+lhh%0D%0Ahhl+lll+hlh+hll+%3B+hhl+lll+lhl+hhh
+","Pleiades Sector OD-S b4-1
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+JM-W+d1-52&data=hll+%3B+hhl+lll+lhl%0D%0Alhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lll+llh+hll+%3B+hhl+lll+lhl+hhh
+",It has some cave-ins. Also got another Thargoid visitor on exiting the site although it left as soon as it arrived.,5,"Yes, unclear where or how many.",4,,
+11/09/2019 02:55:54,ByteSturm,Pleiades Sector LX-U c2-2,AB 4 A,https://i.imgur.com/Vsvtbqo.png,,4,No.,Yes,Pleiades Sector XE-Q b5-2 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+LX-U+c2-2&data=hll+%3B+llh+hlh%0D%0Ahlh+lhl+%3B+hll+hhl+hhh%0D%0Ahll+llh+hll+%3B+hll+lll+hlh,"Result: list index out of range
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+LX-U+c2-2&data=hhh%0D%0Ahhl+hlh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl
+",,It has some cave-ins.,1,"Yes, but unclear where and how many.",4,,
+11/09/2019 02:57:13,ByteSturm,Pleiades Sector JC-V c2-9,1 A,https://i.imgur.com/GGPhNb0.jpg,,4,"Yes, unclear how many and where.",Yes,12 Trianguli https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+JC-V+c2-9&data=hlh+hhh+lhl+%3B+lll+llh+lhh%0D%0Alhh+hlh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhl+lll+hll+%3B+hhl+lll+lhl+hhh,"HIP 12099
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+JC-V+c2-9&data=llh+lhl+%3B+hll+lll+hlh%0D%0Ahll+hll+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhh+llh+lll+%3B+lll+llh+lhh
+","Result: The three spheres do not intersect!
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+JC-V+c2-9&data=hlh+lhl+hll+%3B+lll+llh+lhh%0D%0Alhl+lhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Alhh+lhh+hhl+%3B+hll+lll+hlh
+",It has some cave-ins.,1,"Yes, unclear how many and where.",4,,
+11/09/2019 02:59:20,ByteSturm,Pleiades Sector HH-V c2-13,A 1 C,https://i.imgur.com/5lfZS4B.jpg,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Alnath https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+HH-V+c2-13&data=lhh+lhl+lhl+%3B+hhl+lll+lhl+hhh%0D%0Alhh+llh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhh+lhh+lll+%3B+lll+llh+lhh,"Pemepatung
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+HH-V+c2-13&data=hlh+llh+lhl+%3B+lll+llh+lhh%0D%0Allh+hhh+%3B+hhl+lll+lhl%0D%0Ahll+hlh+hll+%3B+hll+hhl+hhh
+",,It has cave-ins.,4,"Yes, though unclear where or how many.",4,,
+11/09/2019 03:02:03,ByteSturm,Pleiades Sector KT-Q b5-3,5 A,https://i.imgur.com/bEZZRto.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, though unclear where and how many.",Yes,Hyades Sector XO-A c15 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+KT-Q+b5-3&data=hhl+hhh+lll+%3B+lll+llh+lhh%0D%0Allh+hhl+%3B+hll+lll+hlh%0D%0Alll+llh+hll+%3B+lll+llh+lhh,"Pleiades Sector EC-U b3-0
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+KT-Q+b5-3&data=hhl+lhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hll+%3B+lll+llh+lhh%0D%0Ahhl+lhl+lhl+%3B+hhl+lhh+lhh
+","HIP 19026
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+KT-Q+b5-3&data=hll+hll+%3B+hll+lll+hlh%0D%0Ahlh+hhl+%3B+hll+hhl+hhh%0D%0Ahlh+hhh+lhl+lll+%3B+hhl+lll+lhl+hhh
+","Another site down,
+Another off the list.
+Scores more to go,
+I'm starting to feel pissed.",5,No.,5,,
+11/09/2019 03:03:58,ByteSturm,Pleiades Sector EC-U b3-0,4 A,https://i.imgur.com/YmT5ke7.png,,4,"Yes, unclear where and how many.",Yes,Mel 22 Sector NT-Q c5-11 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+EC-U+b3-0&data=lll+lll+%3B+lll+llh+lhh%0D%0Alhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhl+hhl+hhl+%3B+hhl+lll+lhl+hhh,"Pleiades Sector KT-Q b5-3
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+EC-U+b3-0&data=hhl+llh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hll+%3B+lll+llh+lhh%0D%0Ahhl+lhl+lll+%3B+hhl+lhh+lhh
+","Mel 22 Sector OO-Q c5-6
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+EC-U+b3-0&data=hhl+lhl+%3B+hhl+lhh+lhh%0D%0Ahll+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhl+hlh+lll+%3B+hhl+lll+lhl+hhh
+","I drove one eve,
+Long hallowed halls,
+Saw an alien,
+And realized I had no balls.",1,No.,4,,
+11/09/2019 03:05:02,ByteSturm,HIP 15746,AB 1 B,https://imgur.com/a/u4RwuTr,,4,"Yes, unclear where and how many.",Yes,Pleiades Sector EC-U b3-0 https://tool.canonn.tech/linkdecoder/?origin=HIP+15746&data=hhl+lhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hll+%3B+hll+lll+hlh%0D%0Ahhl+lhl+lhl+%3B+hhl+lhh+lhh,"HIP 16704
+https://tool.canonn.tech/linkdecoder/?origin=HIP+15746&data=hll+%3B+lhl+hhh%0D%0Ahhl+%3B+hll+hhl%0D%0Ahll+hhl+hll+%3B+hll+hhl+hhh
+","Result: The three spheres do not intersect!
+https://tool.canonn.tech/linkdecoder/?origin=HIP+15746&data=lhl+lll+%3B+lll+llh+lhh%0D%0Ahhl+llh+%3B+hhl+lll+lhl%0D%0Ahll+hll+hhl+%3B+hll+hhl+hhh
+",Got a Thargoid visitor that simply floated there and observed the site from behind one of the leviathans. Most likely another bugged visit.,1,No.,6,,
+11/09/2019 03:07:02,ByteSturm,Mel 22 Sector SK-E b12-1,2,https://i.imgur.com/tkTsoPI.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, unclear how many and where.",Yes,Mel 22 Sector JC-V d2-15 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+SK-E+b12-1&data=lhh+hhl+%3B+hll+lll+hlh%0D%0Ahlh+lll+%3B+lll+llh+lhh%0D%0Alhh+hhl+lhl+%3B+hll+lll+hlh,"Mel 22 Sector JC-V d2-14
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+SK-E+b12-1&data=hhl+lll+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hll+%3B+hll+lll+hlh%0D%0Ahlh+hhh+llh+lhl+%3B+hhl+lll+lhl+hhh
+","Mel 22 Sector CB-O c6-4
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+SK-E+b12-1&data=hll+lhl+%3B+hll+hhl+hhh%0D%0Alll+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lll+lll+lll+%3B+hhl+lll+lhl+hhh
+","I've come across
+A geological aberration,
+The center of which
+Hides a bright constellation.",5,No.,3,,
+11/09/2019 03:08:15,ByteSturm,Mel 22 Sector JC-V d2-41,A 1 A,https://i.imgur.com/QSRA4p1.png,,4,"Yes, unclear where and how many.",Yes,Etain https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+JC-V+d2-41&data=llh+lhh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhh+hhl+%3B+hll+lll+hlh%0D%0Ahlh+hlh+hll+%3B+hhl+lll+lhl%0D%0A,"Oraon
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+JC-V+d2-41&data=hll+lhl+hhl+%3B+lll+llh+lhh%0D%0Alhh+hll+lll+%3B+lll+llh+lhh%0D%0Ahll+lhl+hhl+%3B+hll+hhl+hhh
+",,"Monotony sets in,
+My SRV feels inert.
+I scan another chamber
+As a Goid egg goes 'splurt'.",1,No.,4,,
+11/09/2019 03:09:33,ByteSturm,Mel 22 Sector JC-V d2-19,4 B,https://i.imgur.com/Q97o8Cd.jpg,Large upright leviathans,4,"Yes, unclear how many and where.",Yes,Pleiades Sector AB-W b2-4 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+JC-V+d2-19&data=hhl+hll+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhl+lhl+%3B+hhl+lll+lhl+hhh%0D%0Alhh+hhh+lll+%3B+hll+lll+hlh,"Kareco
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+JC-V+d2-19&data=hhl+hhl+lhl+%3B+hll+hhl+hhh%0D%0Alll+hll+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhh+llh+hll+%3B+lll+llh+lhh
+",,"Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.",5,No.,3,,
+11/09/2019 03:11:39,ByteSturm,Aries Dark Region HR-W c1-1,8 C,https://i.imgur.com/nLJ8xwl.png,,4,No.,Yes,Pleiades Sector LN-T c3-4 https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+HR-W+c1-1&data=hlh+lhl+%3B+hll+lll+hlh%0D%0Ahhl+hhl+hhl+%3B+lll+llh+lhh%0D%0Ahlh+hhh+lhl+lhl+%3B+hhl+lll+lhl+hhh,"Dulerce
+https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+HR-W+c1-1&data=hhl+hhl+lll+%3B+hll+hhl+hhh%0D%0Ahll+lhl+hhl+%3B+lll+llh+lhh%0D%0Ahlh+hhl+hll+lhl+%3B+hhl+lll+lhl+hhh
+",,It has cave-ins.,1,"Yes, unclear how many and where.",4,,
+11/09/2019 03:12:46,ByteSturm,Mel 22 Sector WK-O c6-1,5 C,https://i.imgur.com/TdV9cs7.jpg,,4,"Yes, unclear how many and where.",Yes,HIP 20644 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+WK-O+c6-1&data=hlh+hlh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahll+lll+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+hll+hll+%3B+hhl+lll+lhl+hhh,"LHS 6187
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+WK-O+c6-1&data=lll+hll+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhh+lll+hll+%3B+hhl+lll+lhl+hhh%0D%0Alhh+lhh+hhl+%3B+hll+lll+hlh
+",,"Monotony sets in,
+My SRV feels inert.
+I scan another chamber
+As a Goid egg goes 'splurt'.",1,No.,5,,
+11/09/2019 03:14:02,ByteSturm,Synuefai YQ-X c17-8,3 A,https://i.imgur.com/DgWkZFN.jpg,,4,"Yes, unclear how many and where.",Yes,Aries Dark Region DB-X c1-2 https://tool.canonn.tech/linkdecoder/?origin=Synuefai+YQ-X+c17-8&data=hhl+hhh+hll+%3B+lll+llh+lhh%0D%0Ahhl+%3B+hll+hhl%0D%0Ahhl+hhh+llh+hhl+%3B+lll+llh+lhh,"Synuefai UA-Y c17-4
+https://tool.canonn.tech/linkdecoder/?origin=Synuefai+YQ-X+c17-8&data=hll+lll+%3B+hll+hhl+hhh%0D%0Ahlh+hll+%3B+hll+lll+hlh%0D%0Ahlh+hlh+hhh+%3B+hhl+lll+lhl
+","Synuefai YQ-X c17-7
+https://tool.canonn.tech/linkdecoder/?origin=Synuefai+YQ-X+c17-8&data=hhl+hlh+hll+%3B+lll+llh+lhh%0D%0Ahhl+lhl+%3B+lll+llh+lhh%0D%0Ahll+lhh+lhl+%3B+hll+hhl+hhh
+","I've started a race
+To get through this place
+Before a random Goid
+Scatters my ship to the void.",1,No.,4,,
+11/09/2019 03:15:46,ByteSturm,Synuefai YQ-X c17-7,1 A,https://i.imgur.com/Oz3CDru.jpg,Large upright leviathans,4,"Yes, unclear how many and where.",Yes,Aries Dark Region HR-W d1-44 https://tool.canonn.tech/linkdecoder/?origin=Synuefai+YQ-X+c17-7&data=hhl+hhl+hhl+%3B+lll+llh+lhh%0D%0Ahll+hll+%3B+hll+lll+hlh%0D%0Ahhl+hll+%3B+hhl+hlh,"Synuefai YQ-X c17-8
+https://tool.canonn.tech/linkdecoder/?origin=Synuefai+YQ-X+c17-7&data=hhl+hhl+lll+%3B+lll+llh+lhh%0D%0Ahhl+lhl+%3B+lll+llh+lhh%0D%0Ahlh+hhl+lhl+%3B+hhl+lll+lhl
+","Result: The three spheres do not intersect!
+https://tool.canonn.tech/linkdecoder/?origin=Synuefai+YQ-X+c17-7&data=hhl+hlh+hll+%3B+lll+llh+lhh%0D%0Ahlh+%3B+hhl+lll+lhl+%0D%0Ahlh+hlh+hhh+%3B+hhl+lll+lhl
+","My engine's humming,
+My heartbeat's thrumming,
+The LZ's not clear,
+The Goids draw near!",5,No.,3,,
+11/09/2019 03:17:20,ByteSturm,Synuefai WV-X c17-12,2 B,https://i.imgur.com/VsqST0L.jpg,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Synuefai YQ-X c17-8 https://tool.canonn.tech/linkdecoder/?origin=Synuefai+WV-X+c17-12&data=hhl+hhl+lll+%3B+lll+llh+lhh%0D%0Ahhl+%3B+hll+hhl%0D%0Ahlh+hhl+lhl+%3B+hhl+lll+lhl,"Pleiades Sector KT-Q b5-3
+https://tool.canonn.tech/linkdecoder/?origin=Synuefai+WV-X+c17-12&data=hhl+llh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+lll+%3B+hll+lll+hlh%0D%0Ahhl+lhl+lll+%3B+hhl+lhh+lhh
+","Synuefai YQ-X c17-7
+https://tool.canonn.tech/linkdecoder/?origin=Synuefai+WV-X+c17-12&data=hhl+hlh+hll+%3B+lll+llh+lhh%0D%0Ahlh+%3B+hhl+lll+lhl%0D%0Ahll+lhh+lhl+%3B+hll+hhl+hhh
+",It has cave-ins.,5,"Yes, unclear how many and where.",4,,
+11/09/2019 03:19:01,ByteSturm,Mel 22 Sector LN-T d3-60,5 A,https://i.imgur.com/NExjWOU.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, unclear where and how many.",Yes,Synuefai WV-X c17-12 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+LN-T+d3-60&data=hhl+hlh+hll+%3B+lll+llh+lhh%0D%0Alhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hlh+hhh+%3B+hhl+lll+lhl,"Synuefai YQ-X c17-7
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+LN-T+d3-60&data=hhl+hlh+hll+%3B+lll+llh+lhh%0D%0Alhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahll+lhh+lhl+%3B+hll+hhl+hhh
+","Aries Dark Region DB-X c1-2
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+LN-T+d3-60&data=hhl+hhh+hll+%3B+lll+llh+lhh%0D%0Ahhl+%3B+lhl+hhh%0D%0Ahhl+hhh+llh+hhl+%3B+lll+llh+lhh
+",It has cave-ins.,4,"Yes, unclear where and how many.",4,,
+11/09/2019 03:20:27,ByteSturm,Aries Dark Region DB-X c1-2,B 1,https://i.imgur.com/8DKpN2s.png,,4,"Yes, unclear where and how many.",Yes,Mel 22 Sector WK-O c6-1 https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+DB-X+c1-2&data=lhh+lll+%3B+hll+lll+hlh%0D%0Ahhl+lhl+%3B+hll+hhl+hhh%0D%0Ahhl+lhl+lll+%3B+hhl+lhh+lhh,"Mel 22 Sector LN-T d3-60
+https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+DB-X+c1-2&data=hlh+hhh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+%3B+lhl+hhh+%0D%0Ahlh+hhl+hll+%3B+hhl+lll+lhl
+","Aries Dark Region DB-X c1-7
+https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+DB-X+c1-2&data=lll+lll+%3B+lll+llh+lhh%0D%0Ahhl+hll+%3B+lll+llh+lhh%0D%0Ahlh+hhl+hll+hhl+%3B+hhl+lll+lhl+hhh
+","It has cave-ins. Was ambushed by a rude pirate leaving the site and lost some hull and items but made it out.
+",1,"Yes, unclear where and how many.",4,,
+11/09/2019 03:22:25,ByteSturm,Aries Dark Region DB-X c1-7,A 9 A,https://i.imgur.com/NK9qiVs.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, unclear where and how many.",Yes,Mel 22 Sector LN-T d3-60 https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+DB-X+c1-7&data=hlh+hhh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Alhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhl+hll+%3B+hhl+lll+lhl,"Aries Dark Region AQ-Y d14
+https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+DB-X+c1-7&data=hll+lll+%3B+hll+lll+hlh%0D%0Ahlh+hll+%3B+lll+llh+lhh%0D%0Ahhl+hhh+lhl+lll+%3B+lll+llh+lhh
+","Aries Dark Region DB-X c1-2
+https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+DB-X+c1-7&data=hhl+hhh+hll+%3B+lll+llh+lhh%0D%0Ahhl+hll+%3B+lll+llh+lhh%0D%0Ahhl+hhh+llh+hhl+%3B+lll+llh+lhh
+","It has cave-ins.
+",3,"Yes, unclear where and how many.",4,,
+11/09/2019 03:23:39,ByteSturm,Synuefai UA-Y c17-4,7 A,https://i.imgur.com/9K81Fjd.jpg,,4,"Yes, unclear where and how many.",Yes,Aries Dark Region HR-W d1-54 https://tool.canonn.tech/linkdecoder/?origin=Synuefai+UA-Y+c17-4&data=hll+%3B+hlh+lhh%0D%0Ahlh+hhh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhl+hhh+hhl+%3B+hhl+lll+lhl+hhh,"Mel 22 Sector LN-T d3-64
+https://tool.canonn.tech/linkdecoder/?origin=Synuefai+UA-Y+c17-4&data=hlh+hll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhl+%3B+hll+hhl+hhh%0D%0Ahll+lhh+lhl+%3B+hll+hhl+hhh
+","Synuefai WV-X c17-12
+https://tool.canonn.tech/linkdecoder/?origin=Synuefai+UA-Y+c17-4&data=hhl+hlh+hll+%3B+lll+llh+lhh%0D%0Alhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hlh+hhh+%3B+hhl+lll+lhl
+","Flying through the dark
+The sight before me is stark.
+Spires litter the ground
+Of the next site I have found.",1,No.,3,,
+11/09/2019 03:25:03,ByteSturm,Mel 22 Sector LN-T d3-64,ABC 1 C,https://i.imgur.com/b7g7Czr.png,,4,"Yes, unclear where and how many.",Yes,Mel 22 Sector OO-Q c5-10 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+LN-T+d3-64&data=hlh+lhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Allh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhl+lll+%3B+hhl+lll+lhl,"Synuefai UA-Y c17-4
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+LN-T+d3-64&data=hll+lll+%3B+hll+hhl+hhh%0D%0Ahhl+hhl+%3B+hll+hhl+hhh%0D%0Ahlh+hlh+hhh+%3B+hhl+lll+lhl
+","Mel 22 Sector OO-Q c5-6
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+LN-T+d3-64&data=hhl+lhl+%3B+hhl+lhh+lhh%0D%0Alhh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhl+hlh+lll+%3B+hhl+lll+lhl+hhh
+","Flitting red eyes
+Flit through the night,
+Droning 'long
+The halls of this site.",1,No.,5,,
+12/09/2019 22:07:25,ByteSturm,Mel 22 Sector OO-Q c5-6,1 A,https://i.imgur.com/mgrd7Di.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, unclear where and how many.",Yes,12 Trianguli https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+OO-Q+c5-6&data=hlh+hhh+lhl+%3B+lll+llh+lhh%0D%0Ahhl+hhl+lhl+%3B+hll+lll+hlh%0D%0Ahlh+hhl+lll+hll+%3B+hhl+lll+lhl+hhh,"Result: Tascheter Sector CL-Y d115 Match is not proven transcript may be wrong
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+OO-Q+c5-6&data=lhl+hlh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Allh+hlh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhl+llh+hhl+%3B+hhl+lll+lhl+hhh
+",,"Another site down,
+Another off the list.
+Scores more to go,
+I'm starting to feel pissed.",3,No.,5,,
+12/09/2019 22:08:59,ByteSturm,Mel 22 Sector OO-Q c5-10,7 B,https://i.imgur.com/3ZdFAZh.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, unclear where and how many.",Yes,Mel 22 Sector OO-Q c5-6 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+OO-Q+c5-10&data=hhl+lhl+%3B+hhl+lhh+lhh%0D%0Ahhl+lhl+%3B+hll+lll+hlh%0D%0Ahlh+hhl+hlh+lll+%3B+hhl+lll+lhl+hhh,"Mel 22 Sector LN-T d3-64
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+OO-Q+c5-10&data=hlh+hll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Allh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+lhh+lhl+%3B+hll+hhl+hhh
+","Mel 22 Sector FM-V d2-67
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+OO-Q+c5-10&data=lhl+hhl+%3B+hll+lll+hlh%0D%0Alhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhl+llh+%3B+hhl+lll+lhl
+","Hippity hoppity,
+The aliens wants me off their property.",3,No.,5,,
+12/09/2019 22:12:47,ByteSturm,Mel 22 Sector FM-V d2-67,2 A,https://i.imgur.com/6wXnmbR.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, unclear where and how many.",Yes,HIP 16795 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+FM-V+d2-67&data=hhl+hhh+hll+%3B+lll+llh+lhh%0D%0Ahhl+hhh+lhl+%3B+lll+llh+lhh%0D%0Ahlh+hhh+hhl+%3B+hhl+lll+lhl,"63 G. Capricorni
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+FM-V+d2-67&data=lll+hll+%3B+hhl+lll+lhl%0D%0Alhh+hhh+lll+%3B+lll+llh+lhh%0D%0Ahlh+hll+hlh+lhl+%3B+hhl+lll+lhl+hhh
+",,"I drove one eve,
+Long hallowed halls,
+Saw an alien,
+And realized I had no balls.",5,No.,4,,
+12/09/2019 22:15:12,ByteSturm,HIP 14969,CD 2 A,https://i.imgur.com/sOfj8m7.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, unclear where and how many.",Yes,Synuefai UZ-M d8-63 https://tool.canonn.tech/linkdecoder/?origin=HIP+14969&data=hlh+hhl+%3B+hhl+lhh+lhh%0D%0Alll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hlh+hlh+hhl+%3B+hhl+lll+lhl+hhh,"Col 285 Sector BQ-X c1-10
+https://tool.canonn.tech/linkdecoder/?origin=HIP+14969&data=hlh+lhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Alll+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hlh+hhh+hhl+%3B+hhl+lll+lhl+hhh
+","Synuefai RK-Y c17-3
+https://tool.canonn.tech/linkdecoder/?origin=HIP+14969&data=hlh+hll+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+%3B+lhl+hhh%0D%0Ahlh+hhl+lll+hll+%3B+hhl+lll+lhl+hhh
+","It has cave-ins (update to the way I fill this out, it has a single cave-in by an exterior door.)",4,"Yes, 1 by a(n exterior) door.",4,,
+12/09/2019 22:16:55,ByteSturm,Mel 22 Sector NT-Q c5-11,8 A,https://i.imgur.com/QR9FmmX.png,,4,"Yes, unclear where and how many.",Yes,HIP 14756 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+NT-Q+c5-11&data=hhl+llh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hll+%3B+hhl+lll+lhl%0D%0Ahll+hll+lll+%3B+hll+hhl+hhh,"Mel 22 Sector FM-V d2-38
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+NT-Q+c5-11&data=hlh+hhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhh+lhl+hhl+%3B+lll+llh+lhh
+","Mel 22 Sector ZM-H b11-3
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+NT-Q+c5-11&data=hhl+hhh+hll+%3B+lll+llh+lhh%0D%0Ahlh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhh+lhh+hll+%3B+lll+llh+lhh
+","Some miles driven,
+Some fuel spent,
+THE EMPEROR APPROVES,
+AND I SHALL MAKE THE XENOS REPENT!",1,No.,4,,
+12/09/2019 22:18:41,ByteSturm,Mel 22 Sector ZM-H b11-3,B 3,https://i.imgur.com/14oDODL.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, unclear where and how many.",Yes,Result: The three spheres do not intersect! https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+ZM-H+b11-3&data=lhl+hhl+%3B+hll+lll+hlh%0D%0Ahlh+lhl+%3B+lll+llh+lhh%0D%0Ahlh+hhl+llh+%3B+hhl+lll+lhl,"Mel 22 Sector FM-V d2-38
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+ZM-H+b11-3&data=hlh+hhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhh+lhl+hhl+%3B+lll+llh+lhh
+","Mel 22 Sector NT-Q c5-11
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+ZM-H+b11-3&data=lll+lll+%3B+lll+llh+lhh%0D%0Ahlh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhl+hhl+hhl+%3B+hhl+lll+lhl+hhh
+","
+Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.",5,No.,5,,
+12/09/2019 22:22:58,ByteSturm,Mel 22 Sector FM-V d2-38,A 3 A,https://i.imgur.com/rDY7rrb.png,,4,"Yes, unclear how many and where.",Yes,HIP 24007 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+FM-V+d2-38&data=hhl+hhh+lll+%3B+lll+llh+lhh%0D%0Ahhl+%3B+lhl%0D%0Ahlh+lll+lll+%3B+hll+hhl+hhh,"Punuri
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+FM-V+d2-38&data=hhl+hll+lhl+%3B+hll+hhl+hhh%0D%0Alll+lhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+lhh+hlh+%3B+hhl+lll+lhl
+",,"Monotony sets in,
+My SRV feels inert.
+I scan another chamber
+As a Goid egg goes 'splurt'.",1,No.,3,,
+12/09/2019 22:24:52,ByteSturm,Pleiades Sector BG-X c1-14,5 D,https://i.imgur.com/FrupOcA.jpg,,4,"Yes, 1 in the tunnels.",Yes,Mel 22 Sector FM-V d2-38 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+BG-X+c1-14&data=hlh+hhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Alhl+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhh+lhl+hhl+%3B+lll+llh+lhh,"Pleiades Sector UZ-X b1-2
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+BG-X+c1-14&data=hhl+lhl+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+lll+%3B+lll+llh+lhh%0D%0Ahlh+hhh+hlh+hhl+%3B+hhl+lll+lhl+hhh
+","Pleiades Sector BG-X c1-10
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+BG-X+c1-14&data=hhl+lhh+hll+%3B+hhl+lll+lhl+hhh%0D%0Alll+%3B+hll+lll+hlh%0D%0Ahlh+hhh+hll+%3B+hhl+lll+lhl
+","Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.",1,It has cave-ins by 1 door and in the tunnels.,4,,
+12/09/2019 22:27:19,ByteSturm,Pleiades Sector UZ-X b1-2,A 1 C,https://i.imgur.com/gaCzEFO.jpg,No Leviathans,2,No.,No,,,,"As soon as I deployed my SRV I was hit with a message, 'Unknown system disruption.' Not sure why, I had parked far enough away from a door. Nothing else happened. Perhaps a bug?
+",2,,0,,
+12/09/2019 22:29:33,ByteSturm,Pleiades Sector BG-X c1-10,14 A,https://i.imgur.com/Q8UYjCg.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Pleiades Sector JM-W d1-52 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+BG-X+c1-10&data=hll+%3B+llh+hlh%0D%0Ahlh+hhl+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lll+lhl+lll+%3B+hhl+lll+lhl+hhh,"Jambin
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+BG-X+c1-10&data=hlh+hhl+hhl+%3B+hll+lll+hlh%0D%0Ahlh+lll+%3B+lhl+hhh%0D%0Ahlh+hll+lll+%3B+hhl+lll+lhl
+",,"I've started a race
+To get through this place
+Before a random Goid
+Scatters my ship to the void.",2,No.,3,,
+12/09/2019 22:31:20,ByteSturm,Pleiades Sector IX-S b4-1,10 A,https://i.imgur.com/mUTxgl6.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 internal door in the tunnels.",Yes,HIP 22382 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+IX-S+b4-1&data=hhl+lll+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hll+%3B+hll+hhl+hhh%0D%0Alll+llh+hhl+%3B+lll+llh+lhh,"Pleiades Sector DG-X c1-14
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+IX-S+b4-1&data=hhl+lhh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+%3B+hll+hhl%0D%0Alll+lhh+lll+%3B+lll+llh+lhh
+","HIP 23022
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+IX-S+b4-1&data=lll+lll+%3B+lll+llh+lhh%0D%0Allh+%3B+hhl+lll+lhl%0D%0Ahll+llh+lll+%3B+hll+lll+hlh
+","Void shrouded by darkness,
+Nothing survives, nothing remains.
+Suddenly LIGHT, COLOR, PIERCING, PARADING!
+LAUGHING AT THE DARKNESS!
+A single ship, it breaks apart.
+Now its twin shines blue.
+They're speaking but one's listening.
+The twin cries out, yelling, screaming voices.
+Silence, persisting, pervading.
+The ship sputters closer, grabs its sibling.
+Engines roar, a course is plotted,
+The ship departs.
+Void shrouded by darkness,
+Nothing survives, nothing remains.",5,It has a cave-in by 1 exterior door and 1 in the tunnels.,4,,
+12/09/2019 22:33:30,ByteSturm,Pleiades Sector DG-X c1-11,A 3A,https://i.imgur.com/PzvDgbU.jpg,Small Reposing Leviathans,2,No.,No,,,,"Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.",4,,1,,
+12/09/2019 22:36:46,ByteSturm,Pleiades Sector DG-X c1-14,AB 1 A,https://i.imgur.com/qq2Z2sd.jpg,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,HIP 21348 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+DG-X+c1-14&data=hhl+hll+%3B+hhl+lll+lhl%0D%0Ahlh+hll+%3B+lll+llh+lhh%0D%0Ahhl+llh+llh+%3B+hhl+lll+lhl,"HIP 24934
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+DG-X+c1-14&data=hlh+lhl+%3B+hhl+lll+lhl%0D%0Ahhl+lhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lhh+lhl+%3B+hhl+lhh+lhh
+","HIP 22334
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+DG-X+c1-14&data=lll+hhl+%3B+lll+llh+lhh%0D%0Alhh+%3B+hhl+lll+lhl%0D%0Ahhl+llh+lhl+lll+%3B+hhl+lll+lhl+hhh
+","I've started a race
+To get through this place
+Before a random Goid
+Scatters my ship to the void.",4,It has a cave-in by 1 exterior door and 1 in the tunnels.,4,,
+12/09/2019 22:40:33,ByteSturm,HIP 24007,1 B,https://i.imgur.com/Izu7f3D.jpg,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,HIP 22382 https://tool.canonn.tech/linkdecoder/?origin=HIP+24007&data=hhl+lll+hhl+%3B+hhl+lll+lhl+hhh%0D%0Allh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Alll+llh+hhl+%3B+lll+llh+lhh,"Result: The three spheres do not intersect!
+https://tool.canonn.tech/linkdecoder/?origin=HIP+24007&data=lll+hll+%3B+lll+llh+lhh%0D%0Ahll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lll+hlh+%3B+hhl+lll+lhl
+","Result: The three spheres do not intersect!
+https://tool.canonn.tech/linkdecoder/?origin=HIP+24007&data=hhl+lll+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+%3B+hhl+lll+lhl%0D%0Ahhl+lll+hhl+hll+%3B+hhl+lll+lhl+hhh
+","Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.",5,It has a cave-in by 1 exterior door and 1 in the tunnels.,4,,
+12/09/2019 22:43:17,ByteSturm,Synuefe LD-A c17-1,B 1 C,https://i.imgur.com/qE98ohg.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Col 285 Sector IL-X c1-4 https://tool.canonn.tech/linkdecoder/?origin=Synuefe+LD-A+c17-1&data=hlh+lhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hlh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+lhl+lhl+%3B+hll+lll+hlh,"HIP 25274
+https://tool.canonn.tech/linkdecoder/?origin=Synuefe+LD-A+c17-1&data=hlh+lhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Alll+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahll+lhl+lll+%3B+hll+lll+hlh
+","Synuefe LD-A c17-3
+https://tool.canonn.tech/linkdecoder/?origin=Synuefe+LD-A+c17-1&data=lll+hll+%3B+lll+llh+lhh%0D%0Ahll+%3B+lll+llh+lhh%0D%0Ahhl+lll+hlh+%3B+hhl+lll+lhl
+","Flitting red eyes
+Flit through the night,
+Droning 'long
+The halls of this site.",5,It has a cave-in by 1 exterior door.,4,,
+12/09/2019 22:45:41,ByteSturm,Synuefe LD-A c17-3,AB 2 F,https://i.imgur.com/FTekx2u.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Pleiades Sector IM-V c2-16 https://tool.canonn.tech/linkdecoder/?origin=Synuefe+LD-A+c17-3&data=hhl+lhh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+lll+%3B+lll+llh+lhh%0D%0Alll+hll+lhl+%3B+lll+llh+lhh,"Result: The three spheres do not intersect!
+https://tool.canonn.tech/linkdecoder/?origin=Synuefe+LD-A+c17-3&data=hlh+lhl+%3B+hll+hhl+hhh%0D%0Ahhl+%3B+hll+hhl%0D%0Ahhl+llh+llh+%3B+hhl+lll+lhl
+","Synuefe LD-A c17-1
+https://tool.canonn.tech/linkdecoder/?origin=Synuefe+LD-A+c17-3&data=hhl+lll+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+%3B+lll+llh+lhh%0D%0Ahhl+lll+hhl+hll+%3B+hhl+lll+lhl+hhh
+","I've come across
+A geological aberration,
+The center of which
+Hides a bright constellation.",4,It has a cave-in by 1 exterior door and 1 in the tunnels.,4,,
+12/09/2019 22:47:17,ByteSturm,HIP 22382,5 E,https://i.imgur.com/7KCpBPQ.jpg,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Col 285 Sector CV-Y d71 https://tool.canonn.tech/linkdecoder/?origin=HIP+22382&data=hhl+hhh+lll+%3B+lll+llh+lhh%0D%0Alhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lll+lll+hhl+%3B+hhl+lll+lhl+hhh,"Col 285 Sector CV-Y d57
+https://tool.canonn.tech/linkdecoder/?origin=HIP+22382&data=hhl+lll+hll+%3B+hhl+lll+lhl+hhh%0D%0Alhh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+hhh+lll+%3B+hll+hhl+hhh
+","HIP 23022
+https://tool.canonn.tech/linkdecoder/?origin=HIP+22382&data=lll+lll+%3B+lll+llh+lhh%0D%0Alll+%3B+hll+lll+hlh%0D%0Ahll+llh+lll+%3B+hll+lll+hlh
+","Flying through the dark
+The sight before me is stark.
+Spires litter the ground
+Of the next site I have found.",4,It has a cave-in by 1 exterior door and 1 in the tunnels.,4,,
+12/09/2019 22:49:35,ByteSturm,HIP 23022,B 2 A,https://i.imgur.com/rr7VoXw.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Col 285 Sector YO-Z c19 https://tool.canonn.tech/linkdecoder/?origin=HIP+23022&data=hlh+lhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Alll+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhh+hhh+hll+%3B+hhl+lll+lhl+hhh,"Result: The three spheres do not intersect!
+https://tool.canonn.tech/linkdecoder/?origin=HIP+23022&data=hhl+lll+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhl+%3B+lll+llh+lhh%0D%0Ahhl+lll+hhl+hll+%3B+hhl+lll+lhl+hhh
+","Synuefe LD-A c17-3
+https://tool.canonn.tech/linkdecoder/?origin=HIP+23022&data=lll+hll+%3B+lll+llh+lhh%0D%0Ahlh+%3B+hhl+lll+lhl+%0D%0Ahhl+lll+hlh+%3B+hhl+lll+lhl%0D%0A","I've come across
+A geological aberration,
+The center of which
+Hides a bright constellation.",5,It has a cave-in by 1 exterior door.,4,,
+12/09/2019 22:52:24,ByteSturm,Col 285 Sector CV-Y d71,5 D,https://i.imgur.com/NT45nRa.jpg,Small Reposing Leviathans,2,No.,No,,,,"Monotony sets in,
+My SRV feels inert.
+I scan another chamber
+As a Goid egg goes 'splurt'.",4,,2,,
+19/11/2019 05:56:01,ByteSturm,Col 285 Sector ZZ-Y d22,2 A,https://i.imgur.com/JRSTYvZ.jpg,Small Reposing Leviathans,2,No.,No,,,,"Some miles driven,
+Some fuel spent,
+THE EMPEROR APPROVES,
+AND I SHALL MAKE THE XENOS REPENT!",5,N/A,2,,
+19/11/2019 05:57:38,ByteSturm,Col 285 Sector YO-Z c19,B 2 A,https://i.imgur.com/veSY95f.jpg,Small Reposing Leviathans,2,No.,No,,,,"I drove one eve,
+Long hallowed halls,
+Saw an alien,
+And realized I had no balls.",5,N/A,2,,
+19/11/2019 05:59:17,ByteSturm,HIP 24934,A 3 A,https://i.imgur.com/HTY1RkZ.jpg,Small Reposing Leviathans,2,No.,No,,,,"Another site down,
+Another off the list.
+Scores more to go,
+I'm starting to feel pissed.",5,N/A,1,,
+20/11/2019 00:41:39,ByteSturm,Wregoe RA-W c15-18,A 1 A,https://i.imgur.com/1AGieD5.jpg,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,HIP 16217 https://tool.canonn.tech/linkdecoder/?origin=Wregoe+RA-W+c15-18&data=lll+%3B+hll+hhl+hhh%0D%0Alhh+lll+%3B+hll+hhl+hhh%0D%0Ahlh+hhh+hhh+lll+%3B+hhl+lll+lhl+hhh,"HIP 16217
+https://tool.canonn.tech/linkdecoder/?origin=Wregoe+RA-W+c15-18&data=lll+%3B+hll+hhl+hhh%0D%0Alhh+lll+%3B+hll+hhl+hhh%0D%0Ahlh+hhh+hhh+lll+%3B+hhl+lll+lhl+hhh
+",,"Hippity hoppity,
+The aliens wants me off their property.",5,Yes. It has a cave-in by 1 exterior door and 1 in the tunnels.,4,,
+20/11/2019 00:43:05,ByteSturm,HIP 23269,A 1 C,https://i.imgur.com/DVKWTwZ.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Wregoe RA-W c15-18 https://tool.canonn.tech/linkdecoder/?origin=HIP+23269&data=lhl+hll+%3B+hll+lll+hlh%0D%0Ahll+%3B+llh+hlh%0D%0Ahhl+lll+hhl+hll+%3B+hhl+lll+lhl+hhh,"Wredguia VX-L d7-80
+https://tool.canonn.tech/linkdecoder/?origin=HIP+23269&data=hlh+lhh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahll+hll+%3B+lll+llh+lhh%0D%0Ahlh+hhh+hll+lhl+%3B+hhl+lll+lhl+hhh
+","Taurus Dark Region DB-X d1-26
+https://tool.canonn.tech/linkdecoder/?origin=HIP+23269&data=lhh+%3B+hll+hhl%0D%0Alll+%3B+lll+llh+lhh%0D%0Ahhl+lll+llh+lhl+%3B+hhl+lll+lhl+hhh
+","Some miles driven,
+Some fuel spent,
+THE EMPEROR APPROVES,
+AND I SHALL MAKE THE XENOS REPENT!",2,Yes. It has a cave-in by 1 exterior door.,4,,
+20/11/2019 00:46:54,ByteSturm,Taurus Dark Region DB-X d1-26,6 E A,https://i.imgur.com/jpAcRbv.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Wregoe RA-W c15-18 https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+DB-X+d1-26&data=lhl+hll+%3B+hll+lll+hlh%0D%0Alll+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lll+hhl+hll+%3B+hhl+lll+lhl+hhh,"Wredguia VX-L d7-80
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+DB-X+d1-26&data=hlh+lhh+hll+%3B+hhl+lll+lhl+hhh%0D%0Alhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhh+hll+lhl+%3B+hhl+lll+lhl+hhh
+","Result: The three spheres do not intersect!
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+DB-X+d1-26&data=hlh+hlh+lll+%3B+hhl+lll+lhl+hhh%0D%0Alll+%3B+lll+llh+lhh%0D%0Ahhl+lll+lhl+hhl+%3B+hhl+lll+lhl+hhh
+","Flitting red eyes
+Flit through the night,
+Droning 'long
+The halls of this site.",3,Yes. It has a cave-in by 1 exterior door and 1 in the tunnels.,4,,
+20/11/2019 00:54:31,ByteSturm,Wredguia VX-L d7-80,5 F,https://i.imgur.com/VrFuqUN.jpg,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Col 285 Sector ZZ-Y d22 https://tool.canonn.tech/linkdecoder/?origin=Wredguia+VX-L+d7-80&data=hlh+hhl+%3B+hhl+lhh+lhh%0D%0Ahhl+hll+%3B+hll+hhl+hhh%0D%0Ahlh+hhh+hll+lhl+%3B+hhl+lll+lhl+hhh,"HIP 23269
+https://tool.canonn.tech/linkdecoder/?origin=Wredguia+VX-L+d7-80&data=hlh+hlh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahll+hll+%3B+lll+llh+lhh%0D%0Ahhl+lll+lhl+hhl+%3B+hhl+lll+lhl+hhh
+","Taurus Dark Region DB-X d1-26
+https://tool.canonn.tech/linkdecoder/?origin=Wredguia+VX-L+d7-80&data=lhh+%3B+hll+hhl%0D%0Alhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lll+llh+lhl+%3B+hhl+lll+lhl+hhh
+","Flying through the dark
+The sight before me is stark.
+Spires litter the ground
+Of the next site I have found.",3,It has a cave-in by 1 exterior door and 1 in the tunnels.,4,,
+20/11/2019 00:58:19,ByteSturm,Wredguia UB-W c15-0,1 A,https://i.imgur.com/dcyjwr9.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Wredguia VX-L d7-80 https://tool.canonn.tech/linkdecoder/?origin=Wredguia+UB-W+c15-0&data=hlh+lhh+hll+%3B+hhl+lll+lhl+hhh%0D%0Alll+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhh+hll+lhl+%3B+hhl+lll+lhl+hhh,"Pleiades Sector BG-X c1-14
+https://tool.canonn.tech/linkdecoder/?origin=Wredguia+UB-W+c15-0&data=hll+lhl+%3B+hll+lll+hlh%0D%0Alll+%3B+hhl+lll+lhl%0D%0Ahlh+hhh+lhl+hll+%3B+hhl+lll+lhl+hhh
+","Mel 22 Sector IS-S c4-6
+https://tool.canonn.tech/linkdecoder/?origin=Wredguia+UB-W+c15-0&data=hhl+hlh+hll+%3B+lll+llh+lhh%0D%0Ahhl+hhl+%3B+hll+lll+hlh%0D%0Ahlh+hhh+lhl+lhl+%3B+hhl+lll+lhl+hhh
+","My engine's humming,
+My heartbeat's thrumming,
+The LZ's not clear,
+The Goids draw near!",2,No.,6,,
+20/11/2019 00:59:19,ByteSturm,HIP 19026,B 1 C,https://i.imgur.com/rtdlg3r.jpg,No Leviathans,2,N/A,No,,,,"I drove one eve,
+Long hallowed halls,
+Saw an alien,
+And realized I had no balls.",4,N/A,0,,
+20/11/2019 01:01:08,ByteSturm,Mel 22 Sector IS-S c4-6,ABC 2 B,https://i.imgur.com/SvWsif3.jpg,Large upright leviathans,4,"Yes, 4 around the central chamber.",Yes,Etain https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+IS-S+c4-6&data=llh+lhh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Alll+hlh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hlh+hll+%3B+hhl+lll+lhl,"Oraon
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+IS-S+c4-6&data=hll+lhl+hhl+%3B+lll+llh+lhh%0D%0Ahll+llh+lll+%3B+lll+llh+lhh%0D%0Ahll+lhl+hhl+%3B+hll+hhl+hhh
+",,"My engine's humming,
+My heartbeat's thrumming,
+The LZ's not clear,
+The Goids draw near!",2,No.,3,,
+20/11/2019 01:02:11,ByteSturm,HIP 22375,3 A,https://i.imgur.com/qZV2CwO.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Opet https://tool.canonn.tech/linkdecoder/?origin=HIP+22375&data=lhh+lll+%3B+hhl+lhh+lhh%0D%0Alll+llh+%3B+hhl+lll+lhl%0D%0Allh+lhl+%3B+llh+hlh,"Iota Pictoris A
+https://tool.canonn.tech/linkdecoder/?origin=HIP+22375&data=llh+lhl+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhh+hll+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lhl+lhl+%3B+hhl+lhh+lhh
+",,"Another site down,
+Another off the list.
+Scores more to go,
+I'm starting to feel pissed.",5,No.,4,,
+20/11/2019 01:03:25,ByteSturm,HIP 21373,2 F,https://i.imgur.com/jCpitT3.jpg,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,HIP 16217 https://tool.canonn.tech/linkdecoder/?origin=HIP+21373&data=lll+%3B+hll+hhl+hhh%0D%0Ahlh+lhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhh+hhh+lll+%3B+hhl+lll+lhl+hhh,"Valkups
+https://tool.canonn.tech/linkdecoder/?origin=HIP+21373&data=lhl+hll+lll+%3B+hhl+lll+lhl+hhh%0D%0Allh+lll+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhl+hhh+hll+%3B+hhl+lll+lhl+hhh
+",,"I drove one eve,
+Long hallowed halls,
+Saw an alien,
+And realized I had no balls.",3,Yes. It has 1 cave-in by an exterior door and 1 in the tunnels.,4,,
+20/11/2019 01:05:30,ByteSturm,HIP 20989,3 C,https://i.imgur.com/jCpitT3.jpg,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Taurus Dark Region BQ-Y c12 https://tool.canonn.tech/linkdecoder/?origin=HIP+20989&data=lhl+hhl+%3B+hll+lll+hlh%0D%0Alhh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+hhh+lhl+%3B+hll+hhl+hhh,"Taurus Dark Region DL-Y d54
+https://tool.canonn.tech/linkdecoder/?origin=HIP+20989&data=hlh+hhh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+llh+hll+%3B+hll+lll+hlh
+","HIP 21373
+https://tool.canonn.tech/linkdecoder/?origin=HIP+20989&data=hlh+hll+%3B+hhl+lll+lhl%0D%0Ahhl+hhl+%3B+lll+llh+lhh%0D%0Ahhl+lll+hhl+hhl+%3B+hhl+lll+lhl+hhh
+","Also saw an NPC Eagle parked near the site. Nothing special, just a planetary NPC spawn. But hey, it was something.",3,Yes. It has a cave-in by 1 exterior door.,4,,
+20/11/2019 01:14:36,ByteSturm,Taurus Dark Region DL-Y d54,ABC 1 C,https://i.imgur.com/i77o7rS.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,HIP 21373 https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+DL-Y+d54&data=hlh+hll+%3B+hhl+lll+lhl%0D%0Ahhl+%3B+hll+hhl%0D%0Ahhl+lll+hhl+hhl+%3B+hhl+lll+lhl+hhh,"Taurus Dark Region BQ-Y c12
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+DL-Y+d54&data=lhl+hhl+%3B+hll+lll+hlh%0D%0Alll+%3B+hll+hhl+hhh%0D%0Ahll+hhh+lhl+%3B+hll+hhl+hhh
+","HIP 20989
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+DL-Y+d54&data=hhl+hhl+lhl+%3B+lll+llh+lhh%0D%0Ahll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+llh+hhl+%3B+hll+lll+hlh
+","Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.",4,Yes. It has a cave-in by 1 exterior door and 1 in the tunnels.,4,,
+20/11/2019 01:21:23,ByteSturm,HIP 20644,A 2 D,https://i.imgur.com/j7SNmJ7.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Taurus Dark Region BV-Y c11 https://tool.canonn.tech/linkdecoder/?origin=HIP+20644&data=lhl+hhl+%3B+hll+lll+hlh%0D%0Alhl+lll+%3B+hhl+lll+lhl+hhh%0D%0Alll+hhl+hll+%3B+lll+llh+lhh,"Hind Sector GR-W d1-13
+https://tool.canonn.tech/linkdecoder/?origin=HIP+20644&data=hlh+lhh+hll+%3B+hhl+lll+lhl+hhh%0D%0Alhh+lhl+%3B+lll+llh+lhh%0D%0Alll+hlh+hll+%3B+lll+llh+lhh
+","Taurus Dark Region QN-T b3-0
+https://tool.canonn.tech/linkdecoder/?origin=HIP+20644&data=hll+lhl+%3B+hll+hhl+hhh%0D%0Ahhl+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+hlh+hhl+%3B+hhl+lll+lhl+hhh
+","
+Monotony sets in,
+My SRV feels inert.
+I scan another chamber
+As a Goid egg goes 'splurt'.",4,No.,5,,
+20/11/2019 01:23:23,ByteSturm,Taurus Dark Region QN-T b3-0,1 B,https://i.imgur.com/91s0xIL.png,Large upright leviathans,4," Yes, 4 around the central structure.",Yes,Taurus Dark Region BV-Y c11 https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+QN-T+b3-0&data=lhl+hhl+%3B+hll+lll+hlh%0D%0Alhl+lll+%3B+hhl+lll+lhl+hhh%0D%0Alll+hhl+hll+%3B+lll+llh+lhh,"HIP 19665
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+QN-T+b3-0&data=hlh+hhl+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+%3B+hll+hhl%0D%0Ahhl+llh+hlh+%3B+hhl+lll+lhl
+","HIP 20644
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+QN-T+b3-0&data=hlh+hlh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+hll+hll+%3B+hhl+lll+lhl+hhh
+","I've come across
+A geological aberration,
+The center of which
+Hides a bright constellation.",5,No.,4,,
+20/11/2019 01:30:44,ByteSturm,Taurus Dark Region BV-Y c11,8 A,https://i.imgur.com/N8j79he.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central structure.",Yes,HIP 20644 https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+BV-Y+c11&data=hlh+hlh+hll+%3B+hhl+lll+lhl+hhh%0D%0Alhl+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+hll+hll+%3B+hhl+lll+lhl+hhh,"Taurus Dark Region QN-T b3-0
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+BV-Y+c11&data=hll+lhl+%3B+hll+hhl+hhh%0D%0Alhl+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+hlh+hhl+%3B+hhl+lll+lhl+hhh
+","Taurus Dark Region CQ-Y d31
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+BV-Y+c11&data=hhl+hhl+hhl+%3B+lll+llh+lhh%0D%0Alhh+%3B+hhl+lll+lhl%0D%0Alll+hlh+lhl+%3B+lll+llh+lhh
+","Flitting red eyes
+Flit through the night,
+Droning 'long
+The halls of this site.",3,No.,6,,
+20/11/2019 01:34:55,ByteSturm,Taurus Dark Region CQ-Y d31,3 C,https://i.imgur.com/SoCEbij.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Pleiades Sector ZZ-Y c14 https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+CQ-Y+d31&data=hhl+hll+%3B+hhl+lhh+lhh%0D%0Ahlh+lhl+%3B+lll+llh+lhh%0D%0Ahhl+llh+llh+%3B+hhl+lll+lhl,"Result: The three spheres do not intersect!
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+CQ-Y+d31&data=hhl+hlh+hhl+%3B+lll+llh+lhh%0D%0Alhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lll+hhl+hll+%3B+hhl+lll+lhl+hhh
+","Taurus Dark Region BV-Y c11
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+CQ-Y+d31&data=lhl+hhl+%3B+hll+lll+hlh+%0D%0Alhh+%3B+hhl+lll+lhl%0D%0Alll+hhl+hll+%3B+lll+llh+lhh
+","Monotony sets in,
+My SRV feels inert.
+I scan another chamber
+As a Goid egg goes 'splurt'.",2,Yes. It has a cave-in by an exterior door.,4,,
+20/11/2019 01:43:27,ByteSturm,Pleiades Sector AV-Y c7,1 B,https://i.imgur.com/65WpHkt.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,HIP 19026 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+AV-Y+c7&data=hll+hll+%3B+hll+lll+hlh%0D%0Ahhl+hhh+hll+%3B+lll+llh+lhh%0D%0Ahlh+hhh+lhl+lll+%3B+hhl+lll+lhl+hhh,"Dhanhopi
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+AV-Y+c7&data=lll+hhh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Alll+lll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhl+lhh+hhl+%3B+lll+llh+lhh
+",,"Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.",5,No.,4,,
+20/11/2019 01:45:30,ByteSturm,Taurus Dark Region QO-Q b5-0,B 2 A,https://i.imgur.com/Qcryklh.png,Large upright leviathans,4," Yes, 4 around the central chamber.",Yes,Taurus Dark Region FW-W c1-3 https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+QO-Q+b5-0&data=hhl+%3B+hhl+hhh%0D%0Ahll+%3B+llh+hlh%0D%0Ahhl+lll+lll+hhl+%3B+hhl+lll+lhl+hhh,"HIP 22375
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+QO-Q+b5-0&data=hhl+hlh+hhl+%3B+lll+llh+lhh%0D%0Allh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lll+hhl+hll+%3B+hhl+lll+lhl+hhh
+","Pleiades Sector AV-Y c7
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+QO-Q+b5-0&data=hhl+lhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hll+%3B+lll+llh+lhh%0D%0Ahhl+lll+hhh+hll+%3B+hhl+lll+lhl+hhh
+","I've started a race
+To get through this place
+Before a random Goid
+Scatters my ship to the void.",2,No.,3,,
+26/11/2019 17:12:09,ByteSturm,Pleiades Sector EB-X c1-2,ABC 3 C,https://i.imgur.com/Qcryklh.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,HIP 20859 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+EB-X+c1-2&data=hhl+hlh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Alll+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+lhl+lhl+%3B+hhl+lll+lhl+hhh,"Pleiades Sector AV-Y c7
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+EB-X+c1-2&data=hhl+lhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Alll+%3B+hhl+lll+lhl%0D%0Ahhl+lll+hhh+hll+%3B+hhl+lll+lhl+hhh
+","HIP 17862 (?)
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+EB-X+c1-2&data=hhl+hll+%3B+lll+llh+lhh%0D%0Ahlh+lhl+%3B+lll+llh+lhh%0D%0Ahhl+lll+lhh+hhl+%3B+hhl+lll+lhl+hhh
+","I drove one eve,
+Long hallowed halls,
+Saw an alien,
+And realized I had no balls.",2,"Yes. It has 1 cave-in by an exterior door and 2 cave-ins in the tunnels. Yes, it has more than 1 on the interior this time.",4,,
+26/11/2019 17:15:01,ByteSturm,HIP 20859,A 1 A,https://i.imgur.com/D0NPpsZ.png,Large upright leviathans,4,"Yes, 4 around the central chamber.",Yes,HIP 16613 https://tool.canonn.tech/linkdecoder/?origin=HIP+20859&data=llh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+hll+%3B+hll+lll+hlh%0D%0Ahhl+lll+hlh+lll+%3B+hhl+lll+lhl+hhh,"Agastani
+https://tool.canonn.tech/linkdecoder/?origin=HIP+20859&data=llh+lhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Alll+hhh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hlh+hlh+%3B+hhl+lll+lhl
+",,"Some miles driven,
+Some fuel spent,
+THE EMPEROR APPROVES,
+AND I SHALL MAKE THE XENOS REPENT!",3,No.,4,,
+26/11/2019 17:17:28,ByteSturm,Pleiades Sector EL-Y d23,ABCD 1 A,https://i.imgur.com/tP56EJo.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Pleiades Sector AV-Y c7 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+EL-Y+d23&data=hhl+lhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+lhl+%3B+lll+llh+lhh%0D%0Ahhl+lll+hhh+hll+%3B+hhl+lll+lhl+hhh,"Pleiades Sector IX-S b4-1
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+EL-Y+d23&data=hhl+hlh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+%3B+hhl+hlh%0D%0Ahhl+lll+lhl+hll+%3B+hhl+lll+lhl+hhh
+","HIP 20859
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+EL-Y+d23&data=hhl+hlh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Alll+%3B+hll+hhl+hhh%0D%0Ahhl+llh+lhl+lhl+%3B+hhl+lll+lhl+hhh
+",Had a Thargoid visitor after exiting the site. This one actually destroyed my ship since I lifted off before the energy surge so it was able to scan me and as it proceeded to bump into the terrain it got mad at me for not releasing cargo and turned red and pissy. Did not witness any Thargons deploy.,3,No.,5,,
+02/12/2019 02:15:15,ByteSturm,Pleiades Sector ZZ-Y c14,A 1 A,https://i.imgur.com/eek8NhN.png,"Large upright leviathans, Small Reposing Leviathans",4," Yes, 4 around the central chamber.",Yes,Pleiades Sector AV-Y c7 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+ZZ-Y+c14&data=hhl+lhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhl+%3B+hll+lll+hlh%0D%0Ahhl+lll+hhh+hll+%3B+hhl+lll+lhl+hhh,"Pleiades Sector IX-S b4-1
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+ZZ-Y+c14&data=hhl+hlh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+lhl+%3B+hll+lll+hlh%0D%0Ahhl+lll+lhl+hll+%3B+hhl+lll+lhl+hhh
+","Taurus Dark Region JH-V c2-9
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+ZZ-Y+c14&data=hll+lll+%3B+hll+lll+hlh%0D%0Ahll+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+llh+lll+%3B+hhl+lll+lhl+hhh
+","Hippity hoppity,
+The aliens wants me off their property.",3,No.,6,,
+02/12/2019 02:18:14,ByteSturm,Taurus Dark Region CQ-Y d36,7 A,https://i.imgur.com/5AmHUAv.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Pleiades Sector ZZ-Y c14 https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+CQ-Y+d36&data=hhl+hll+%3B+hhl+lhh+lhh%0D%0Alll+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+llh+%3B+hhl+lll+lhl,"Taurus Dark Region CQ-Y d31
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+CQ-Y+d36&data=hhl+hhl+hhl+%3B+lll+llh+lhh%0D%0Ahhl+hll+%3B+hll+hhl+hhh%0D%0Alll+hlh+lhl+%3B+lll+llh+lhh
+","HIP 22743
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+CQ-Y+d36&data=lll+lll+%3B+lll+llh+lhh%0D%0Ahhl+hhl+%3B+hll+hhl+hhh%0D%0Ahhl+llh+hll+lll+%3B+hhl+lll+lhl+hhh
+","Another site down,
+Another off the list.
+Scores more to go,
+I'm starting to feel pissed.",5,No.,4,,
+02/12/2019 02:20:37,ByteSturm,HIP 22743,B 1 A,https://i.imgur.com/aFCZ93j.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Bhal https://tool.canonn.tech/linkdecoder/?origin=HIP+22743&data=hll+lll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Alhh+lll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Alhh+hll+lll+%3B+hll+lll+hlh,"LDS 883
+https://tool.canonn.tech/linkdecoder/?origin=HIP+22743&data=lhl+hll+lll+%3B+hhl+lll+lhl+hhh%0D%0Allh+hhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hlh+hhl+hhl+%3B+hhl+lll+lhl+hhh
+",,"My engine's humming,
+My heartbeat's thrumming,
+The LZ's not clear,
+The Goids draw near!",3,No.,6,,
+02/12/2019 02:21:51,ByteSturm,Taurus Dark Region JH-V c2-9,AB 1 A,https://i.imgur.com/TyeKdMn.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the outer tunnels.",Yes,HIP 22743 https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+JH-V+c2-9&data=lll+lll+%3B+lll+llh+lhh%0D%0Ahll+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+hll+lll+%3B+hhl+lll+lhl+hhh,"Pleiades Sector ZZ-Y c14
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+JH-V+c2-9&data=hhl+hll+%3B+hhl+lhh+lhh%0D%0Ahll+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+llh+%3B+hhl+lll+lhl
+","Taurus Dark Region CQ-Y d36
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+JH-V+c2-9&data=hhl+hhl+lll+%3B+lll+llh+lhh%0D%0Alll+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lhl+lll+lhl+%3B+hhl+lll+lhl+hhh
+","I've come across
+A geological aberration,
+The center of which
+Hides a bright constellation.",3,Yes. It has a cave-in by 1 exterior door and 1 cave-in in the tunnels.,4,,
+02/12/2019 03:00:08,ByteSturm,HIP 22334,1 D,https://i.imgur.com/bXOdnZ7.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,HIP 21348 https://tool.canonn.tech/linkdecoder/?origin=HIP+22334&data=hhl+hll+%3B+hhl+lll+lhl%0D%0Ahll+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+llh+%3B+hhl+lll+lhl,"Taurus Dark Region JH-V c2-9
+https://tool.canonn.tech/linkdecoder/?origin=HIP+22334&data=hll+lll+%3B+hll+lll+hlh%0D%0Ahlh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+llh+lll+%3B+hhl+lll+lhl+hhh
+","Result: The three spheres do not intersect!
+https://tool.canonn.tech/linkdecoder/?origin=HIP+22334&data=lll+lll+%3B+lll+llh+lhh%0D%0Ahlh+%3B+hhl+lll+lhl%0D%0Ahhl+llh+hll+lll+%3B+hhl+lll+lhl+hhh
+","Monotony sets in,
+My SRV feels inert.
+I scan another chamber
+As a Goid egg goes 'splurt'.",3,No.,5,,
+02/12/2019 03:05:18,ByteSturm,HIP 21348,A 2 B,https://i.imgur.com/HCtayBL.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Result: The three spheres do not intersect! https://tool.canonn.tech/linkdecoder/?origin=HIP+21348&data=hhl+lhh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hll+%3B+lll+llh+lhh%0D%0Alll+lhh+lll+%3B+lll+llh+lhh,"HIP 22334
+https://tool.canonn.tech/linkdecoder/?origin=HIP+21348&data=lll+hhl+%3B+lll+llh+lhh%0D%0Ahll+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+lhl+lll+%3B+hhl+lll+lhl+hhh
+","HIP 20859
+https://tool.canonn.tech/linkdecoder/?origin=HIP+21348&data=hhl+hlh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+lhl+lhl+%3B+hhl+lll+lhl+hhh
+","Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.",3,No.,4,,
+02/12/2019 04:56:37,ByteSturm,HIP 22118,B 3 A,https://i.imgur.com/nR3FfYP.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Pleiades Sector KH-V c2-7 https://tool.canonn.tech/linkdecoder/?origin=HIP+22118&data=hhl+lhl+lhl+%3B+hhl+lll+lhl+hhh%0D%0Alhh+%3B+hhl+lll+lhl%0D%0Ahhl+llh+lhl+hll+%3B+hhl+lll+lhl+hhh,"HIP 17044
+https://tool.canonn.tech/linkdecoder/?origin=HIP+22118&data=lll+%3B+hhl+lhh+lhh%0D%0Ahll+lhl+%3B+hll+lll+hlh%0D%0Ahhl+lhh+lll+%3B+hhl+lhh+lhh
+","Pleiades Sector GW-W d1-65
+https://tool.canonn.tech/linkdecoder/?origin=HIP+22118&data=llh+hll+%3B+lll+llh+lhh%0D%0Alll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+llh+hll+%3B+hhl+lll+lhl+hhh
+","I've started a race
+To get through this place
+Before a random Goid
+Scatters my ship to the void.",5,Yes. It has 1 cave-in in the tunnels and 1 cave-in by a door.,4,,
+02/12/2019 04:58:18,ByteSturm,Synuefe HZ-M d8-69,B 7 F,https://i.imgur.com/te3pu1s.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Pleiades Sector PN-T b3-0 https://tool.canonn.tech/linkdecoder/?origin=Synuefe+HZ-M+d8-69&data=lll+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+llh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lll+hlh+hll+%3B+hhl+lll+lhl+hhh,"RMK 6
+https://tool.canonn.tech/linkdecoder/?origin=Synuefe+HZ-M+d8-69&data=lll+hhh+hll+%3B+hhl+lll+lhl+hhh%0D%0Allh+hhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhh+lhl+hhl+%3B+lll+llh+lhh
+",,"Flitting red eyes
+Flit through the night,
+Droning 'long
+The halls of this site.",3,Yes. It has 2 cave-ins in the tunnels and 1 cave-in by a door.,4,,
+02/12/2019 04:59:21,ByteSturm,Pleiades Sector KH-V c2-7,7 F,https://i.imgur.com/pTWuAqd.png,No Leviathans,2,N/A,No,,,,"I've come across
+A geological aberration,
+The center of which
+Hides a bright constellation.",5,N/A,0,,
+02/12/2019 05:00:41,ByteSturm,Pleiades Sector IM-V c2-16,ABC 2 A,https://i.imgur.com/6q3384o.png,Small Reposing Leviathans,2,N/A,No,,,,"Flying through the dark
+The sight before me is stark.
+Spires litter the ground
+Of the next site I have found.",4,N/A,1,,
+02/12/2019 05:01:36,ByteSturm,Pleiades Sector GW-W d1-65,10 F,https://i.imgur.com/cWQ6wWS.png,No Leviathans,2,N/A,No,,,,"Some miles driven,
+Some fuel spent,
+THE EMPEROR APPROVES,
+AND I SHALL MAKE THE XENOS REPENT!",5,N/A,0,,
+02/12/2019 05:02:59,ByteSturm,Taurus Dark Region OX-U c2-10,4 B,https://i.imgur.com/DZ2ZLbb.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Result: The three spheres do not intersect! https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+OX-U+c2-10&data=lll+lhl+%3B+lll+llh+lhh%0D%0Alhh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Allh+llh+lll+%3B+lll+llh+lhh,"Result: The three spheres do not intersect!
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+OX-U+c2-10&data=lhl+hhh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+llh+hhl+%3B+hll+hhl+hhh
+","HIP 21251
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+OX-U+c2-10&data=lll+lhl+%3B+lll+llh+lhh%0D%0Ahlh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahll+hll+lll+%3B+hll+lll+hlh
+","My engine's humming,
+My heartbeat's thrumming,
+The LZ's not clear,
+The Goids draw near!",3,No.,4,,
+09/12/2019 22:42:19,ByteSturm,HIP 21251,A 8 C,https://i.imgur.com/CJG5nTO.png,Large upright leviathans,4,"Yes, 4 around the central chamber.",Yes,Outotz PX-J b51-0 (?) https://tool.canonn.tech/linkdecoder/?origin=HIP+21251&data=hhl+hlh+llh+lhl+%3B+lll+llh+lhh%0D%0Ahlh+hll+lll+%3B+hhl+lll+lhl%0D%0Ahhl+lll+hhl+%3B+hhl+lll+lhl,"Outotz QJ-O c20-0 (?)
+https://tool.canonn.tech/linkdecoder/?origin=HIP+21251&data=hhl+lhh+hll+lll+%3B+lll+llh+lhh%0D%0Ahlh+lll+hhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhh+lhl+lhl+%3B+lll+llh+lhh
+",,"Another site down,
+Another off the list.
+Scores more to go,
+I'm starting to feel pissed.",5,No.,4,,
+09/12/2019 22:44:02,ByteSturm,Taurus Dark Region IR-W d1-47,A 3 A,https://i.imgur.com/VLuoUPn.png,Large upright leviathans,4,"Yes, 4 around the central chamber.",Yes,Struve's Lost Sector NN-T c3-6 https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+IR-W+d1-47&data=lhh+hhl+%3B+hll+lll+hlh%0D%0Ahlh+lhl+%3B+lll+llh+lhh%0D%0Ahhl+lhl+lhl+%3B+hhl+lll+lhl,"Taurus Dark Region OX-U c2-10
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+IR-W+d1-47&data=hll+lll+%3B+hll+lll+hlh%0D%0Ahll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Allh+lll+hhl+%3B+lll+llh+lhh
+","HIP 21251
+https://tool.canonn.tech/linkdecoder/?origin=Taurus+Dark+Region+IR-W+d1-47&data=lll+lhl+%3B+lll+llh+lhh%0D%0Ahhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahll+hll+lll+%3B+hll+lll+hlh
+","Hippity hoppity,
+The aliens wants me off their property.
+",5,No.,3,,
+09/12/2019 22:46:46,ByteSturm,Struve's Lost Sector NN-T c3-6,1 C,https://i.imgur.com/X9E6qcs.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Taurus Dark Region OX-U c2-10 https://tool.canonn.tech/linkdecoder/?origin=Struve%27s+Lost+Sector+NN-T+c3-6&data=hll+lll+%3B+hll+lll+hlh%0D%0Alhh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Allh+lll+hhl+%3B+lll+llh+lhh,"HIP 20318
+https://tool.canonn.tech/linkdecoder/?origin=Struve%27s+Lost+Sector+NN-T+c3-6&data=hlh+hlh+lll+%3B+hhl+lll+lhl+hhh%0D%0Alhh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lhl+hlh+hll+%3B+hhl+lll+lhl+hhh
+","Result: The three spheres do not intersect!
+https://tool.canonn.tech/linkdecoder/?origin=Struve%27s+Lost+Sector+NN-T+c3-6&data=lll+lhl+%3B+lll+llh+lhh%0D%0Ahll+hll+%3B+hhl+lll+lhl+hhh%0D%0Allh+llh+lll+%3B+lll+llh+lhh
+","I drove one eve,
+Long hallowed halls,
+Saw an alien,
+And realized I had no balls.",2,No.,4,,
+09/12/2019 22:49:01,ByteSturm,Struve's Lost Sector NN-T c3-1,ABCD 1 A,https://i.imgur.com/Rph5MEA.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Pleiades Sector JN-S b4-3 https://tool.canonn.tech/linkdecoder/?origin=Struve%27s+Lost+Sector+NN-T+c3-1&data=lll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hlh+lll+%3B+lll+llh+lhh%0D%0Ahlh+lhl+%3B+hlh+lhh,"Di Jian
+https://tool.canonn.tech/linkdecoder/?origin=Struve%27s+Lost+Sector+NN-T+c3-1&data=hhl+%3B+hlh%0D%0Ahlh+hhl+hll+%3B+hll+lll+hlh%0D%0Ahlh+hll+lhl+lll+%3B+hhl+lll+lhl+hhh
+",,"I've come across
+A geological aberration,
+The center of which
+Hides a bright constellation.",5,No.,5,,
+09/12/2019 22:52:27,ByteSturm,HIP 20830,A 8 F,https://i.imgur.com/4OBzKWi.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Struve's Lost Sector NN-T c3-6 https://tool.canonn.tech/linkdecoder/?origin=HIP+20830&data=lhh+hhl+%3B+hll+lll+hlh%0D%0Ahhl+hhl+%3B+hll+hhl+hhh%0D%0Ahhl+lhl+lhl+%3B+hhl+lll+lhl,"Result: The three spheres do not intersect!
+https://tool.canonn.tech/linkdecoder/?origin=HIP+20830&data=lll+lhl+%3B+lll+llh+lhh%0D%0Ahll+%3B+hhl+lhh+lhh%0D%0Allh+llh+lll+%3B+lll+llh+lhh
+","HIP 20318
+https://tool.canonn.tech/linkdecoder/?origin=HIP+20830&data=hlh+hlh+lll+%3B+hhl+lll+lhl+hhh%0D%0Alll+%3B+hll+lll+hlh%0D%0Ahhl+lhl+hlh+hll+%3B+hhl+lll+lhl+hhh
+","Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.",4,Yes. It has 2 cave-ins in the tunnels and 1 cave-in by a door.,4,,
+09/12/2019 22:55:15,ByteSturm,HIP 20318,3 A,https://i.imgur.com/HKIYddQ.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Result: The three spheres do not intersect! https://tool.canonn.tech/linkdecoder/?origin=HIP+20318&data=lll+lhl+%3B+lll+llh+lhh%0D%0Alll+%3B+hll+hhl+hhh%0D%0Allh+llh+lll+%3B+lll+llh+lhh,"Struve's Lost Sector NN-T c3-6
+https://tool.canonn.tech/linkdecoder/?origin=HIP+20318&data=lhh+hhl+%3B+hll+lll+hlh%0D%0Alhh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lhl+lhl+%3B+hhl+lll+lhl
+","Result: The three spheres do not intersect!
+https://tool.canonn.tech/linkdecoder/?origin=HIP+20318&data=hlh+hlh+hll+%3B+hhl+lll+lhl+hhh%0D%0Alll+%3B+hll+lll+hlh%0D%0Ahhl+lhl+hlh+lhl+%3B+hhl+lll+lhl+hhh+
+","I've started a race
+To get through this place
+Before a random Goid
+Scatters my ship to the void.",3,No.,4,,
+09/12/2019 23:19:58,ByteSturm,HIP 18778,A 2 A,https://i.imgur.com/c0hus2U.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Struve's Lost Sector OI-T c3-3 https://tool.canonn.tech/linkdecoder/?origin=HIP+18778&data=lll+lhl+%3B+lll+llh+lhh%0D%0Ahll+hll+%3B+lll+llh+lhh%0D%0Alll+hhh+lhl+%3B+lll+llh+lhh,"HIP 20318
+https://tool.canonn.tech/linkdecoder/?origin=HIP+18778&data=hlh+hlh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+%3B+hlh+lhh%0D%0Ahhl+lhl+hlh+hll+%3B+hhl+lll+lhl+hhh
+","HIP 18117
+https://tool.canonn.tech/linkdecoder/?origin=HIP+18778&data=hhl+hhl+lhl+%3B+lll+llh+lhh%0D%0Alhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+hll+lhl+%3B+hll+lll+hlh
+","Monotony sets in,
+My SRV feels inert.
+I scan another chamber
+As a Goid egg goes 'splurt'.",5,No.,4,,
+09/12/2019 23:24:37,ByteSturm,HIP 19266,6 A,https://i.imgur.com/8l5pXTF.png,Large upright leviathans,4,"Yes, 4 around the central chamber.",Yes,Mel 22 Sector DG-O c6-0 https://tool.canonn.tech/linkdecoder/?origin=HIP+19266&data=hhl+hhl+lll+%3B+lll+llh+lhh%0D%0Ahhl+lhl+%3B+hll+hhl+hhh+%0D%0Ahhl+hhl+%3B+hhl+hlh,"Result: The three spheres do not intersect!
+https://tool.canonn.tech/linkdecoder/?origin=HIP+19266&data=hlh+hlh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lhl+%3B+hll+hhl+hhh%0D%0Ahhl+lhl+hlh+lhl+%3B+hhl+lll+lhl+hhh
+","Mel 22 Sector MC-V d2-19
+https://tool.canonn.tech/linkdecoder/?origin=HIP+19266&data=hhl+hhl+lll+%3B+lll+llh+lhh%0D%0Ahlh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lhl+lhh+%3B+hhl+lll+lhl
+","Flying through the dark
+The sight before me is stark.
+Spires litter the ground
+Of the next site I have found.",5,No.,4,,
+09/12/2019 23:27:57,ByteSturm,Mel 22 Sector MC-V d2-19,BC 1 B,https://i.imgur.com/pH2hhCB.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Ngaiawang https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+MC-V+d2-19&data=hhl+hlh+hll+%3B+hll+hhl+hhh%0D%0Allh+lhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhl+hlh+lhl+%3B+hhl+lll+lhl+hhh,"Wayutabal
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+MC-V+d2-19&data=hhl+hll+%3B+hll+hhl%0D%0Ahhl+lhl+lhl+%3B+hll+lll+hlh%0D%0Ahhl+hhh+hll+lll+%3B+lll+llh+lhh
+",,"My engine's humming,
+My heartbeat's thrumming,
+The LZ's not clear,
+The Goids draw near!",3,No.,5,,
+09/12/2019 23:31:50,ByteSturm,Mel 22 Sector BV-P c5-2,1 A,https://i.imgur.com/TZDnTCh.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,HIP 16217 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+BV-P+c5-2&data=lll+%3B+hll+hhl+hhh%0D%0Ahlh+llh+%3B+hhl+lll+lhl%0D%0Ahlh+hhh+hhh+lll+%3B+hhl+lll+lhl+hhh,"HIP 30045
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+BV-P+c5-2&data=llh+hll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+lhh+lll+%3B+lll+llh+lhh%0D%0Ahlh+hhh+lhh+hll+%3B+hhl+lll+lhl+hhh
+",,"I drove one eve,
+Long hallowed halls,
+Saw an alien,
+And realized I had no balls.",5,No.,5,,
+10/12/2019 01:52:27,ByteSturm,Mel 22 Sector YU-F b11-1,AB 5 A,https://i.imgur.com/nNeWaQs.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Outotz SU-W b57-0 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+YU-F+b11-1&data=hhl+hhh+lll+hll+%3B+lll+llh+lhh%0D%0Ahhl+hhh+llh+lll+%3B+lll+llh+lhh%0D%0Ahhl+lhl+hhh+lhl+%3B+hhl+lll+lhl+hhh,"Outotz EH-M d7-5
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+YU-F+b11-1&data=hhl+lhh+hll+lhl+%3B+lll+llh+lhh%0D%0Ahhl+hhl+lll+%3B+llh+hlh%0D%0Ahhl+hll+hhl+lll+%3B+lll+llh+lhh
+",,"Had a Thargoid visitor. I decided to stay in my SRV this time and see what it did, but first I transferred my probe and sensor to my ship JUST in case. In the end, I think that saved my life, as this Thargoid didn't bounce around the surface, but actually approached and scanned me in my SRV. Since I had nothing it wanted, it promptly left without scanning my ship that was sitting close by.
+",5,No.,6,,
+10/12/2019 01:54:08,ByteSturm,Mel 22 Sector UT-R c4-4,1 A,https://i.imgur.com/g517uzq.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Synuefai UA-Y c17-4 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+UT-R+c4-4&data=hll+lll+%3B+hll+hhl+hhh%0D%0Ahlh+hhh+hll+%3B+lll+llh+lhh%0D%0Ahlh+hlh+hhh+%3B+hhl+lll+lhl,"HR 3499
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+UT-R+c4-4&data=hhl+hlh+hhl+%3B+hll+hhl+hhh%0D%0Ahhl+hhh+hhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hlh+hhl+hhl+%3B+hhl+lll+lhl+hhh
+",,"My engine's humming,
+My heartbeat's thrumming,
+The LZ's not clear,
+The Goids draw near!",4,Yes. It has 1 cave-in in the tunnels and 1 cave-in by a(n exterior) door.,4,,
+10/12/2019 01:58:24,ByteSturm,HIP 18117,5 D,https://i.imgur.com/NwVde4Q.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,HIP 18778 https://tool.canonn.tech/linkdecoder/?origin=HIP+18117&data=hhl+hhl+lll+%3B+lll+llh+lhh%0D%0Alhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Alhl+hll+%3B+llh+hlh,"HIP 18117
+(Points to same site.)","Struve's Lost Sector OI-T c3-3
+https://tool.canonn.tech/linkdecoder/?origin=HIP+18117&data=lll+lhl+%3B+lll+llh+lhh%0D%0Alhh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Alll+hhh+lhl+%3B+lll+llh+lhh
+","The second audio clip recovered from this site activated the link and had it pointing to the same site.
+",3,Yes. It has 1 cave-in in the tunnels and 1 cave-in by a(n exterior) door.,4,,
+10/12/2019 02:01:39,ByteSturm,Mel 22 Sector UE-G b11-0,A 2,https://i.imgur.com/WlkFHWW.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Aries Dark Region DB-X d1-64 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+UE-G+b11-0&data=hhl+hhh+hll+%3B+lll+llh+lhh%0D%0Ahll+llh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+hll+hll+%3B+hll+hhl+hhh,"Ross 129
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+UE-G+b11-0&data=lll+lhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhl+hhh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hlh+llh+hll+%3B+hhl+lll+lhl+hhh
+",,"Flying through the dark
+The sight before me is stark.
+Spires litter the ground
+Of the next site I have found.",5,No.,4,,
+10/12/2019 02:05:14,ByteSturm,HIP 18730,3 G A,https://i.imgur.com/AkL0g9o.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,HIP 19549 https://tool.canonn.tech/linkdecoder/?origin=HIP+18730&data=hhl+hhl+%3B+hhl+lhh+lhh%0D%0Alhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+hlh+lll+%3B+hhl+lll+lhl+hhh,"HIP 18778
+https://tool.canonn.tech/linkdecoder/?origin=HIP+18730&data=hhl+hhl+lll+%3B+lll+llh+lhh%0D%0Alhh+lll+%3B+lll+llh+lhh%0D%0Alhl+hll+%3B+llh+hlh
+","Pleiades Sector MM-V b2-2
+https://tool.canonn.tech/linkdecoder/?origin=HIP+18730&data=hhl+hhl+%3B+hhl+lhh+lhh%0D%0Ahlh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+hlh+hhl+%3B+hhl+lll+lhl+hhh
+","Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.",3,Yes. It has 1 cave-in in the tunnels and 1 cave-in by a(n exterior) door.,4,,
+10/12/2019 02:08:34,ByteSturm,Pleiades Sector MM-V b2-2,1 B,https://i.imgur.com/iojcA9V.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Pleiades Sector GG-Y d33 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+MM-V+b2-2&data=lhl+lll+%3B+lll+llh+lhh%0D%0Ahlh+hhl+%3B+lll+llh+lhh%0D%0Ahhl+llh+hll+hll+%3B+hhl+lll+lhl+hhh,"HIP 19549
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+MM-V+b2-2&data=hhl+hhl+%3B+hhl+lhh+lhh%0D%0Ahlh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+hlh+lll+%3B+hhl+lll+lhl+hhh
+","HIP 18730
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+MM-V+b2-2&data=hhl+hlh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+hll+hhl+%3B+hhl+lll+lhl+hhh
+","I've started a race
+To get through this place
+Before a random Goid
+Scatters my ship to the void.",3,No.,5,,
+10/12/2019 02:10:56,ByteSturm,HIP 19549,A 2 C,https://i.imgur.com/agnbAEe.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Pleiades Sector DQ-Y c4 https://tool.canonn.tech/linkdecoder/?origin=HIP+19549&data=hhl+lhh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+lll+%3B+lll+llh+lhh%0D%0Alll+hlh+lhl+%3B+lll+llh+lhh,"HIP 18730
+https://tool.canonn.tech/linkdecoder/?origin=HIP+19549&data=hhl+hlh+hll+%3B+hhl+lll+lhl+hhh%0D%0Alhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+hll+hhl+%3B+hhl+lll+lhl+hhh
+","Pleiades Sector MM-V b2-2
+https://tool.canonn.tech/linkdecoder/?origin=HIP+19549&data=hhl+hhl+%3B+hhl+lhh+lhh%0D%0Ahlh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+hlh+hhl+%3B+hhl+lll+lhl+hhh
+","Monotony sets in,
+My SRV feels inert.
+I scan another chamber
+As a Goid egg goes 'splurt'.",3,Yes. It has 1 cave-in by a(n exterior) door.,4,,
+10/12/2019 02:14:09,ByteSturm,Pleiades Sector MC-V c2-0,1 A,https://i.imgur.com/itWNo3v.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,HIP 20785 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+MC-V+c2-0&data=hhl+hlh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhl+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lll+hhh+lll+%3B+hhl+lll+lhl+hhh,"Pleiades Sector MC-V c2-8
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+MC-V+c2-0&data=hhl+%3B+hhl+hlh%0D%0Alhh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+lhh+hhl+%3B+hhl+lll+lhl+hhh
+","Synuefe UZ-X c17-10
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+MC-V+c2-0&data=hhl+hhl+lll+%3B+lll+llh+lhh%0D%0Ahhl+lhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+llh+lll+%3B+hll+hhl+hhh
+","I've come across
+A geological aberration,
+The center of which
+Hides a bright constellation.",4,Yes. It has 2 cave-ins in the tunnels and 1 cave-in by a(n exterior) door.,4,,
+10/12/2019 02:17:58,ByteSturm,Pleiades Sector KH-V c2-3,3 A,https://i.imgur.com/NgHAwJA.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Pleiades Sector HR-W d1-17 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+KH-V+c2-3&data=hlh+lhl+%3B+lll+llh+lhh%0D%0Ahhl+hhl+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhh+hhh+hhl+%3B+lll+llh+lhh,"Wolf 636
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+KH-V+c2-3&data=lll+hll+%3B+hhl+lll+lhl%0D%0Ahhl+hhl+%3B+hlh+lhh%0D%0Ahhl+hhl+lhl+hhl+%3B+lll+llh+lhh
+",,"The site had a specifically blueish tint, but that could be due to the lighting of the surrounding system and planet.
+",4,Yes. It has 1 cave-in in the tunnels and 1 cave-in by a(n exterior) door.,4,,
+10/12/2019 02:20:30,ByteSturm,Pleiades Sector IR-W d1-76,B 3 A,https://i.imgur.com/5CPAMd8.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Pleiades Sector LN-T c3-4 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+IR-W+d1-76&data=hlh+lhl+%3B+hll+lll+hlh%0D%0Alhl+lll+%3B+lll+llh+lhh%0D%0Ahlh+hhh+lhl+lhl+%3B+hhl+lll+lhl+hhh,"CW Ursae Majoris
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+IR-W+d1-76&data=llh+lll+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hlh+hhl+%3B+hll+hhl+hhh%0D%0Allh+%3B+lhl
+",,"Flitting red eyes
+Flit through the night,
+Droning 'long
+The halls of this site.",3,Yes. It has 1 cave-in by a(n exterior) door.,4,,
+10/12/2019 02:21:58,ByteSturm,Pleiades Sector KH-V c2-1,2 A,https://i.imgur.com/A1CSZBj.png,Small Reposing Leviathans,2,N/A,No,,,,"Another site down,
+Another off the list.
+Scores more to go,
+I'm starting to feel pissed.",3,N/A,1,,
+10/12/2019 02:23:54,ByteSturm,HIP 20785,6 B,https://i.imgur.com/ZFqR0RZ.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,HIP 19792 https://tool.canonn.tech/linkdecoder/?origin=HIP+20785&data=lll+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lll+lhh+lll+%3B+hhl+lll+lhl+hhh,"Otherni
+https://tool.canonn.tech/linkdecoder/?origin=HIP+20785&data=lhl+lll+hll+%3B+hhl+lll+lhl+hhh%0D%0Alhh+lll+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhh+lhl+lll+%3B+hhl+lll+lhl+hhh
+",,"Some miles driven,
+Some fuel spent,
+THE EMPEROR APPROVES,
+AND I SHALL MAKE THE XENOS REPENT!",3,No.,5,,
+10/12/2019 02:25:30,ByteSturm,Col 285 Sector CV-Y d57,AB 4 A,https://i.imgur.com/fhx6CN3.png,No Leviathans,2,N/A,No,,,,"Hippity hoppity,
+The aliens wants me off their property.",5,N/A,0,,
+10/12/2019 02:27:34,ByteSturm,HIP 25274,8 A,https://i.imgur.com/joD76ck.png,Large upright leviathans,2,N/A,No,,,,"I drove one eve,
+Long hallowed halls,
+Saw an alien,
+And realized I had no balls.",3,N/A,1,,
+10/12/2019 02:29:23,ByteSturm,Col 285 Sector IL-X c1-4,A 2 C,https://i.imgur.com/kmOoQ8t.png,Small Reposing Leviathans,2,N/A,No,,,,"Some miles driven,
+Some fuel spent,
+THE EMPEROR APPROVES,
+AND I SHALL MAKE THE XENOS REPENT!",3,N/A,2,,
+10/12/2019 02:38:30,ByteSturm,Col 285 Sector IL-X c1-16,A 4 A,https://i.imgur.com/cEm6v9W.png,No Leviathans,2,N/A,No,,,,"I've come across
+A geological aberration,
+The center of which
+Hides a bright constellation.",5,N/A,0,,
+10/12/2019 02:40:16,ByteSturm,HIP 22347,4 A,https://i.imgur.com/IeDSiI7.png,No Leviathans,2,N/A,No,,,,"Flitting red eyes
+Flit through the night,
+Droning 'long
+The halls of this site.",4,N/A,0,,
+10/12/2019 02:42:05,ByteSturm,Hyades Sector XO-A c15,2 A,https://i.imgur.com/VG6YJYA.png,Small Reposing Leviathans,2,N/A,No,,,,"Flying through the dark
+The sight before me is stark.
+Spires litter the ground
+Of the next site I have found.",5,N/A,1,,
+10/12/2019 02:45:19,ByteSturm,HIP 20964,3 B,https://i.imgur.com/fboIknG.png,Small Reposing Leviathans,2,N/A,No,,,,"My engine's humming,
+My heartbeat's thrumming,
+The LZ's not clear,
+The Goids draw near!",5,N/A,1,,
+10/12/2019 02:47:49,ByteSturm,HIP 18909,A 4 E A,https://i.imgur.com/d7GBFR8.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Result: The three spheres do not intersect! https://tool.canonn.tech/linkdecoder/?origin=HIP+18909&data=lll+hhl+%3B+lll+llh+lhh%0D%0Ahll+%3B+hhl+lhh+lhh%0D%0Ahhl+lll+lhl+hhl+%3B+hhl+lll+lhl+hhh,"Aries Dark Region GW-W d1-86
+https://tool.canonn.tech/linkdecoder/?origin=HIP+18909&data=hlh+hlh+hll+%3B+hhl+lll+lhl+hhh%0D%0Alll+%3B+hll+lll+hlh%0D%0Ahhl+lll+llh+hll+%3B+hhl+lll+lhl+hhh
+","HIP 15134
+https://tool.canonn.tech/linkdecoder/?origin=HIP+18909&data=hlh+lhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahll+lll+%3B+lll+llh+lhh%0D%0Alhh+hhh+lll+%3B+hll+lll+hlh
+","I drove one eve,
+Long hallowed halls,
+Saw an alien,
+And realized I had no balls.",3,Yes. It has 2 cave-ins in the tunnels and 1 cave-in by a(n external) door.,4,,
+10/12/2019 02:50:27,ByteSturm,Aries Dark Region GW-W d1-86,1 B,https://i.imgur.com/90XN0k9.png,No Leviathans,2,N/A,No,,,,"Some miles driven,
+Some fuel spent,
+THE EMPEROR APPROVES,
+AND I SHALL MAKE THE XENOS REPENT!",3,N/A,0,,
+10/12/2019 02:51:44,ByteSturm,Aries Dark Region MS-T c3-2,A 1 A,https://i.imgur.com/ND3HQWx.png,Small Reposing Leviathans,2,N/A,No,,,,"Another site down,
+Another off the list.
+Scores more to go,
+I'm starting to feel pissed.",3,N/A,2,,
+10/12/2019 02:53:11,ByteSturm,Hyades Sector PC-V b2-3,2 A,https://i.imgur.com/x1KSGz7.png,Small Reposing Leviathans,2,N/A,No,,,,"Hippity hoppity,
+The aliens wants me off their property.",5,N/A,1,,
+10/12/2019 02:54:53,ByteSturm,Aries Dark Region GW-W d1-48,6 A,https://i.imgur.com/TnzmBoe.png,No Leviathans,2,N/A,No,,,,"I've started a race
+To get through this place
+Before a random Goid
+Scatters my ship to the void.",3,N/A,0,,
+10/12/2019 02:56:37,ByteSturm,Aries Dark Region IM-V c2-5,12 A,https://i.imgur.com/RXnNlgU.png,Small Reposing Leviathans,2,N/A,No,,,,"Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.",3,N/A,1,,
+10/12/2019 03:29:14,ByteSturm,Pleiades Sector GW-W d1-54,2 C,https://i.imgur.com/mOL7S9k.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,HIP 22347 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+GW-W+d1-54&data=hlh+lhh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lll+hlh+hhl+%3B+hhl+lll+lhl+hhh,"Pleiades Sector LS-T c3-2
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+GW-W+d1-54&data=hhl+lhh+%3B+hhl+lll+lhl%0D%0Alll+%3B+hll+hhl+hhh%0D%0Ahlh+hhh+hhh+%3B+hhl+lll+lhl
+","Pleiades Sector OY-R c4-19
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+GW-W+d1-54&data=hhl+lhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhl+%3B+lll+llh+lhh%0D%0Ahlh+hhh+hll+hll+%3B+hhl+lll+lhl+hhh
+","Had a Thargoid visitor that shut down my SRV and then promptly left. Maybe it was due to me being underneath my ship just before it pulsed.
+",3,Yes. It has 1 cave-in by a(n exterior) door.,4,,
+10/12/2019 03:33:02,ByteSturm,Pleiades Sector MC-V c2-8,8 B,https://i.imgur.com/mOL7S9k.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Pleiades Sector MC-V c2-0 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+MC-V+c2-8&data=hhl+hlh+lll+%3B+hhl+lll+lhl+hhh%0D%0Alhh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+lll+%3B+hll+hhl,"HIP 18368 (?)
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+MC-V+c2-8&data=lll+hhl+%3B+lll+llh+lhh%0D%0Ahhl+hhl+%3B+hll+lll+hlh%0D%0Ahhl+llh+llh+%3B+hhl+lll+lhl
+","Synuefe TF-U b36-2 (?)
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+MC-V+c2-8&data=hlh+hll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhl+%3B+hll+lll+hlh%0D%0Alll+hhl+hhl+%3B+lll+llh+lhh
+","The site also has a blueish tint on the inside, could be due to surrounding terrain/system lighting.
+",3,Yes. It has 1 cave-in in the tunnels and 1 cave-in by a(n exterior) door.,4,,
+10/12/2019 03:38:31,ByteSturm,Pleiades Sector MC-V c2-6,B 4 A,https://i.imgur.com/yMzoKHv.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Pleiades Sector MC-V c2-0 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+MC-V+c2-6&data=hhl+hlh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahll+%3B+hhl+lhh+lhh%0D%0Ahlh+lll+%3B+hll+hhl,"Synuefe JU-M d8-50
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+MC-V+c2-6&data=hhl+hhh+lll+%3B+lll+llh+lhh%0D%0Ahhl+hll+%3B+hll+lll+hlh%0D%0Allh+lll+hll+%3B+lll+llh+lhh
+","Pleiades Sector MC-V c2-8
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+MC-V+c2-6&data=hhl+%3B+hhl+hlh%0D%0Ahhl+hhl+%3B+lll+llh+lhh%0D%0Ahhl+llh+lhh+hhl+%3B+hhl+lll+lhl+hhh
+","Monotony sets in,
+My SRV feels inert.
+I scan another chamber
+As a Goid egg goes 'splurt'.",3,Yes. It has 1 cave-in by a(n exterior) door.,4,,
+10/12/2019 03:39:48,ByteSturm,Synuefe WU-X c17-8,2 A,https://i.imgur.com/1dbH0hU.png,Small Reposing Leviathans,2,N/A,No,,,,"Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.",3,N/A,2,,
+10/12/2019 03:41:39,ByteSturm,HIP 20486,A 3 A,https://i.imgur.com/MxDPHQ0.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Synuefe UK-V b35-0 https://tool.canonn.tech/linkdecoder/?origin=HIP+20486&data=hhl+hhl+lhl+%3B+lll+llh+lhh%0D%0Ahlh+lhl+%3B+lll+llh+lhh%0D%0Alll+hhh+hll+%3B+lll+llh+lhh,"Synuefe YP-X c17-1
+https://tool.canonn.tech/linkdecoder/?origin=HIP+20486&data=hlh+hlh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Alhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Alll+hhh+hll+%3B+lll+llh+lhh
+","Result: The three spheres do not intersect!
+https://tool.canonn.tech/linkdecoder/?origin=HIP+20486&data=hlh+hhl+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hll+%3B+lll+llh+lhh%0D%0Ahll+lhh+lhl+%3B+hll+lll+hlh
+","I've come across
+A geological aberration,
+The center of which
+Hides a bright constellation.",5,Yes. It has 1 cave-in in the tunnels and 1 cave-in by a(n exterior) door.,4,,
+10/12/2019 03:43:47,ByteSturm,Synuefe UZ-X c17-12,AB 2 A,https://i.imgur.com/pn38q6E.png,No Leviathans,2,N/A,No,,,,"Flitting red eyes
+Flit through the night,
+Droning 'long
+The halls of this site.",5,N/A,0,,
+10/12/2019 03:49:11,ByteSturm,Synuefe UZ-X c17-10,1 B,https://i.imgur.com/hhhrXIK.png,Small Reposing Leviathans,2,N/A,No,,,,"Found a Thargoid uplink device very close to the main structure sitting on a ruined bit of leviathan. Probably just random spawn, but interesting placement. Also, a System Defence Force ship spawned landed outside of the site. Probably spying on me. >:O
+",3,N/A,1,,
+10/12/2019 03:50:12,ByteSturm,HIP 22851,7 A,https://i.imgur.com/fECVChJ.png,Small Reposing Leviathans,2,N/A,No,,,,"Flying through the dark
+The sight before me is stark.
+Spires litter the ground
+Of the next site I have found.",3,N/A,1,,
+10/12/2019 03:53:37,ByteSturm,Synuefe RT-Z c16-13,1 A,https://i.imgur.com/yJCrLOk.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Pleiades Sector MC-V c2-6 https://tool.canonn.tech/linkdecoder/?origin=Synuefe+RT-Z+c16-13&data=hhl+lhh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahll+%3B+llh+hlh%0D%0Ahhl+llh+hll+hhl+%3B+hhl+lll+lhl+hhh,"HIP 22851
+https://tool.canonn.tech/linkdecoder/?origin=Synuefe+RT-Z+c16-13&data=hlh+hll+hhl+%3B+hhl+lll+lhl+hhh%0D%0Allh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+lhh+hll+%3B+hll+lll+hlh
+","Synuefe JU-M d8-50
+https://tool.canonn.tech/linkdecoder/?origin=Synuefe+RT-Z+c16-13&data=hhl+hhh+lll+%3B+lll+llh+lhh%0D%0Ahhl+hll+%3B+lll+llh+lhh%0D%0Allh+lll+hll+%3B+lll+llh+lhh
+","My engine's humming,
+My heartbeat's thrumming,
+The LZ's not clear,
+The Goids draw near!",3,Yes. It has 1 cave-in by a(n exterior) door and 1 cave-in in the tunnels.,4,,
+10/12/2019 03:59:48,ByteSturm,Synuefe JU-M d8-50,5 A,https://i.imgur.com/yQTvRMO.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,HIP 20486 (?) https://tool.canonn.tech/linkdecoder/?origin=Synuefe+JU-M+d8-50&data=hlh+lhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Allh+%3B+hhl+lll+lhl%0D%0Allh+llh+lll+%3B+lll+llh+lhh,"Pleiades Sector MC-V c2-6
+https://tool.canonn.tech/linkdecoder/?origin=Synuefe+JU-M+d8-50&data=hhl+lhh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hll+%3B+hll+lll+hlh%0D%0Ahhl+llh+hll+hhl+%3B+hhl+lll+lhl+hhh
+","Synuefe RT-Z c16-13
+https://tool.canonn.tech/linkdecoder/?origin=Synuefe+JU-M+d8-50&data=lhh+lll+%3B+hll+lll+hlh%0D%0Ahhl+hll+%3B+lll+llh+lhh%0D%0Ahhl+lhl+lhh+lhl+%3B+hhl+lll+lhl+hhh
+","I drove one eve,
+Long hallowed halls,
+Saw an alien,
+And realized I had no balls.",3,Yes. It has 1 cave-in by a(n exterior) door and 1 cave-in in the tunnels.,4,,
+10/12/2019 04:03:07,ByteSturm,Synuefe YP-X c17-1,5 C,https://i.imgur.com/jkFggEW.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Synuefe YP-X c17-8 https://tool.canonn.tech/linkdecoder/?origin=Synuefe+YP-X+c17-1&data=hhl+hlh+hll+%3B+lll+llh+lhh%0D%0Ahhl+hhl+%3B+hll+lll+hlh%0D%0Ahll+lhh+lhl+%3B+hll+lll+hlh,"Synuefe OV-K d9-56
+https://tool.canonn.tech/linkdecoder/?origin=Synuefe+YP-X+c17-1&data=hlh+hll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lhl+%3B+hll+lll+hlh%0D%0Ahhl+llh+lhl+lll+%3B+hhl+lll+lhl+hhh
+","Synuefe UK-V b35-0
+https://tool.canonn.tech/linkdecoder/?origin=Synuefe+YP-X+c17-1&data=hhl+hhl+lhl+%3B+lll+llh+lhh%0D%0Alll+%3B+hhl+lll+lhl+hhh%0D%0Alll+hhh+hll+%3B+lll+llh+lhh
+","Some miles driven,
+Some fuel spent,
+THE EMPEROR APPROVES,
+AND I SHALL MAKE THE XENOS REPENT!",3,Yes. It has 1 cave-in by a(n exterior) door and 1 cave-in in the tunnels.,4,,
+10/12/2019 18:55:07,ByteSturm,Synuefe UK-V b35-0,A 4 A,https://i.imgur.com/vIal2WC.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Synuefe RT-Z c16-13 https://tool.canonn.tech/linkdecoder/?origin=Synuefe+UK-V+b35-0&data=lhh+lll+%3B+hll+lll+hlh%0D%0Ahlh+lll+%3B+hll+lll+hlh%0D%0Ahhl+lhl+lhh+lhl+%3B+hhl+lll+lhl+hhh,"Synuefe YP-X c17-1
+https://tool.canonn.tech/linkdecoder/?origin=Synuefe+UK-V+b35-0&data=hlh+hlh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Alll+%3B+hhl+lll+lhl+hhh%0D%0Alll+hhh+hll+%3B+lll+llh+lhh
+","Synuefe CW-V c18-6
+https://tool.canonn.tech/linkdecoder/?origin=Synuefe+UK-V+b35-0&data=hlh+lhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Alhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+hll+lll+%3B+hhl+lll+lhl+hhh
+","Another site down,
+Another off the list.
+Scores more to go,
+I'm starting to feel pissed.",5,Yes. It has 1 cave-in by a(n exterior) door and 1 cave-in in the tunnels.,4,,
+10/12/2019 18:56:20,ByteSturm,Synuefe YP-X c17-8,2 A,https://i.imgur.com/mMvuPXO.png,Small Reposing Leviathans,2,N/A,No,,,,"Some miles driven,
+Some fuel spent,
+THE EMPEROR APPROVES,
+AND I SHALL MAKE THE XENOS REPENT!",3,N/A,1,,
+10/12/2019 18:57:36,ByteSturm,Synuefe OV-K d9-56,9 C,https://i.imgur.com/keri5uB.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Aries Dark Region PH-V b2-0 (?) https://tool.canonn.tech/linkdecoder/?origin=Synuefe+OV-K+d9-56&data=lll+hhl+%3B+lll+llh+lhh%0D%0Allh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+llh+%3B+hhl+lll+lhl,"Synuefe CW-V c18-6
+https://tool.canonn.tech/linkdecoder/?origin=Synuefe+OV-K+d9-56&data=hlh+lhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hll+%3B+lll+llh+lhh%0D%0Ahhl+llh+hll+lll+%3B+hhl+lll+lhl+hhh
+","Synuefe YP-X c17-1
+https://tool.canonn.tech/linkdecoder/?origin=Synuefe+OV-K+d9-56&data=hlh+hlh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lhl+%3B+hll+lll+hlh%0D%0Alll+hhh+hll+%3B+lll+llh+lhh
+","Hippity hoppity,
+The aliens wants me off their property.",5,Yes. It has 1 cave-in by a(n exterior) door and 1 cave-in in the tunnels.,4,,
+10/12/2019 18:59:44,ByteSturm,Synuefe CW-V c18-6,A 3 A,https://i.imgur.com/uT3iZ5V.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Synuefe UK-V b35-0 https://tool.canonn.tech/linkdecoder/?origin=Synuefe+CW-V+c18-6&data=hhl+hhl+lhl+%3B+lll+llh+lhh%0D%0Alhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Alll+hhh+hll+%3B+lll+llh+lhh,"Synuefe OV-K d9-56
+https://tool.canonn.tech/linkdecoder/?origin=Synuefe+CW-V+c18-6&data=hlh+hll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hll+%3B+lll+llh+lhh%0D%0Ahhl+llh+lhl+lll+%3B+hhl+lll+lhl+hhh
+","Synuefe YP-X c17-8
+https://tool.canonn.tech/linkdecoder/?origin=Synuefe+CW-V+c18-6&data=hhl+hlh+hll+%3B+lll+llh+lhh%0D%0Alll+%3B+lll+llh+lhh+%0D%0Ahll+lhh+lhl+%3B+hll+lll+hlh
+","I drove one eve,
+Long hallowed halls,
+Saw an alien,
+And realized I had no balls.",3,Yes. It has 1 cave-in by a(n exterior) door and 1 cave-in in the tunnels.,4,,
+10/12/2019 19:01:31,ByteSturm,HIP 15443,AB 4 B,https://i.imgur.com/KQnZyPO.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,HIP 14746 https://tool.canonn.tech/linkdecoder/?origin=HIP+15443&data=hlh+lhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+%3B+lhl+hhh%0D%0Ahhl+llh+llh+%3B+hhl+lll+lhl,"Synuefe MK-M d8-20
+https://tool.canonn.tech/linkdecoder/?origin=HIP+15443&data=hlh+lhl+%3B+hhl+lll+lhl%0D%0Ahll+%3B+hhl+lhh+lhh%0D%0Ahhl+llh+hhl+lll+%3B+hhl+lll+lhl+hhh
+","HIP 14702
+https://tool.canonn.tech/linkdecoder/?origin=HIP+15443&data=hhl+hhl+hhl+%3B+lll+llh+lhh%0D%0Alll+%3B+hhl+lll+lhl%0D%0Allh+hhl+%3B+llh+hlh
+","Monotony sets in,
+My SRV feels inert.
+I scan another chamber
+As a Goid egg goes 'splurt'.",3,Yes. It has 1 cave-in by a(n exterior) door and 2 cave-ins in the tunnels.,4,,
+10/12/2019 19:03:59,ByteSturm,HIP 14746,6 A,https://i.imgur.com/L40VJAX.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Synuefe CW-V c18-6 https://tool.canonn.tech/linkdecoder/?origin=HIP+14746&data=hlh+lhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hlh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+hll+lll+%3B+hhl+lll+lhl+hhh,"Synuefe MK-M d8-20
+https://tool.canonn.tech/linkdecoder/?origin=HIP+14746&data=hlh+lhl+%3B+hhl+lll+lhl+%0D%0Ahhl+hhl+%3B+hll+lll+hlh%0D%0Ahhl+llh+hhl+lll+%3B+hhl+lll+lhl+hhh
+","HIP 15443
+https://tool.canonn.tech/linkdecoder/?origin=HIP+14746&data=hlh+lhh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+%3B+lhl+hhh%0D%0Ahlh+lll+hll+%3B+hll+hhl+hhh","Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.",4,Yes. It has 1 cave-in by a(n exterior) door and 2 cave-ins in the tunnels.,4,,
+10/12/2019 19:05:52,ByteSturm,Synuefe MK-M d8-20,A 2 A,https://i.imgur.com/FPmIcKF.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,HIP 14746 https://tool.canonn.tech/linkdecoder/?origin=Synuefe+MK-M+d8-20&data=hlh+lhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhl+%3B+hll+lll+hlh%0D%0Ahhl+llh+llh+%3B+hhl+lll+lhl,"HIP 15443
+https://tool.canonn.tech/linkdecoder/?origin=Synuefe+MK-M+d8-20&data=hlh+lhh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahll+%3B+hhl+lhh+lhh%0D%0Ahlh+lll+hll+%3B+hll+hhl+hhh
+","Pleiades Sector KM-W d1-40
+https://tool.canonn.tech/linkdecoder/?origin=Synuefe+MK-M+d8-20&data=hhl+hhl+%3B+hhl+lhh+lhh%0D%0Ahhl+hhl+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+lll+lhl+%3B+hll+hhl+hhh
+",Had another Thargoid visitor. This one also left as soon as it pulsed. I believe it's because I was near or underneath my ship shortly before the pulse instead of out in the open.,3,Yes. It has 1 cave-in by a(n exterior) door and 2 cave-ins in the tunnels.,4,,
+10/12/2019 19:08:57,ByteSturm,Mel 22 Sector DG-O c6-0,2 A,https://i.imgur.com/uhB0Qig.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Mel 22 Sector BV-P c5-2 https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+DG-O+c6-0&data=hlh+lhh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lhl+%3B+hll+lll+hlh%0D%0Ahlh+llh+hll+%3B+hll+hhl+hhh,"HIP 15443
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+DG-O+c6-0&data=hlh+lhh+hll+%3B+hhl+lll+lhl+hhh%0D%0Alhl+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+lll+hll+%3B+hll+hhl+hhh
+","Synuefe MK-M d8-20
+https://tool.canonn.tech/linkdecoder/?origin=Mel+22+Sector+DG-O+c6-0&data=hlh+lhl+%3B+hhl+lll+lhl%0D%0Ahhl+hll+%3B+lll+llh+lhh%0D%0Ahhl+llh+hhl+lll+%3B+hhl+lll+lhl+hhh
+","I've started a race
+To get through this place
+Before a random Goid
+Scatters my ship to the void.",3,Yes. It has 1 cave-in by a(n exterior) door.,4,,
+10/12/2019 19:10:45,ByteSturm,Pleiades Sector KM-W d1-40,B 10 A,https://i.imgur.com/xgHeScW.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,HIP 16246 (?) https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+KM-W+d1-40&data=lll+hhl+%3B+lll+llh+lhh%0D%0Alhh+lhl+%3B+lll+llh+lhh%0D%0Ahhl+llh+llh+%3B+hhl+lll+lhl,"Pleiades Sector OS-U c2-7
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+KM-W+d1-40&data=lhl+lll+%3B+lll+llh+lhh%0D%0Ahlh+lll+%3B+lll+llh+lhh%0D%0Ahhl+lll+hlh+hll+%3B+hhl+lll+lhl+hhh
+","HIP 16572
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+KM-W+d1-40&data=lhl+hhl+%3B+lll+llh+lhh%0D%0Ahlh+hll+%3B+lll+llh+lhh%0D%0Ahhl+lll+hhl+hll+%3B+hhl+lll+lhl+hhh
+","I've come across
+A geological aberration,
+The center of which
+Hides a bright constellation.",3,Yes. It has 1 cave-in by a(n exterior) door and 1 cave-in in the tunnels.,4,,
+10/12/2019 19:12:45,ByteSturm,Aries Dark Region JW-W c1-4,7 A,https://i.imgur.com/dc4142g.png,Small Reposing Leviathans,2,N/A,No,,,,"Flitting red eyes
+Flit through the night,
+Droning 'long
+The halls of this site.",3,N/A,2,,
+10/12/2019 19:15:23,ByteSturm,HIP 18368,2 H,https://i.imgur.com/Q2pIhur.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,12 Trianguli https://tool.canonn.tech/linkdecoder/?origin=HIP+18368&data=hlh+hhh+lhl+%3B+lll+llh+lhh%0D%0Ahhl+llh+lll+%3B+lll+llh+lhh%0D%0Ahlh+hhl+lll+hll+%3B+hhl+lll+lhl+hhh,"Byeru Bese
+https://tool.canonn.tech/linkdecoder/?origin=HIP+18368&data=lll+hhh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+hhh+lll+%3B+lll+llh+lhh%0D%0Ahll+lll+hhl+%3B+hll+hhl+hhh
+",,"Flying through the dark
+The sight before me is stark.
+Spires litter the ground
+Of the next site I have found.",3,No.,6,,
+10/12/2019 19:18:58,ByteSturm,Pleiades Sector IR-W d1-38,ABCD 1 A,https://i.imgur.com/I65iIA6.png,No Leviathans,2,N/A,No,,,,"My engine's humming,
+My heartbeat's thrumming,
+The LZ's not clear,
+The Goids draw near!",3,N/A,0,,
+10/12/2019 19:20:36,ByteSturm,Pleiades Sector WE-Q b5-2,1 A,https://i.imgur.com/k8FQkVk.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Pleiades Sector IR-W d1-38 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+WE-Q+b5-2&data=lll+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahll+%3B+hhl+lhh+lhh%0D%0Alll+hll+lll+%3B+lll+llh+lhh,"Result: The three spheres do not intersect!
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+WE-Q+b5-2&data=lhl+hhl+%3B+lll+llh+lhh%0D%0Ahhl+hll+%3B+lll+llh+lhh%0D%0Ahhl+lll+hhl+hll+%3B+hhl+lll+lhl+hhh
+","Pleiades Sector IR-W d1-61
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+WE-Q+b5-2&data=lhh+lhl+%3B+lll+llh+lhh%0D%0Ahlh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+llh+lll+%3B+hll+lll+hlh
+","Some miles driven,
+Some fuel spent,
+THE EMPEROR APPROVES,
+AND I SHALL MAKE THE XENOS REPENT!",3,Yes. There is 1 cave-in by a(n exterior) door and 1 cave-in in the tunnels.,4,,
+10/12/2019 19:21:44,ByteSturm,HIP 16572,A 4 E,https://i.imgur.com/c0VwIOR.png,Small Reposing Leviathans,2,N/A,No,,,,"Experienced some kind of visual glitch when deploying my SRV, probably due to the way that I landed. Screen was going crazy, but had no effect on actual deployment.
+",3,N/A,2,,
+10/12/2019 19:22:55,ByteSturm,Pleiades Sector IR-W d1-61,BC 1 A,https://i.imgur.com/EIMxGHp.png,Small Reposing Leviathans,2,N/A,No,,,,"Void shrouded by darkness,
+Nothing survives, nothing remains.
+Suddenly LIGHT, COLOR, PIERCING, PARADING!
+LAUGHING AT THE DARKNESS!
+A single ship, it breaks apart.
+Now its twin shines blue.
+They're speaking but one's listening.
+The twin cries out, yelling, screaming voices.
+Silence, persisting, pervading.
+The ship sputters closer, grabs its sibling.
+Engines roar, a course is plotted,
+The ship departs.
+Void shrouded by darkness,
+Nothing survives, nothing remains.",3,N/A,2,,
+10/12/2019 19:24:32,ByteSturm,Pleiades Sector MX-U d2-63,1 A,https://i.imgur.com/VESVliL.png,No Leviathans,2,N/A,No,,,,"Monotony sets in,
+My SRV feels inert.
+I scan another chamber
+As a Goid egg goes 'splurt'.",5,N/A,0,,
+10/12/2019 19:26:51,ByteSturm,Aries Dark Region KH-V c2-4,7 A,https://i.imgur.com/SSw2mzc.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Aries Dark Region HR-W d1-34 https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+KH-V+c2-4&data=lll+hhl+%3B+lll+llh+lhh%0D%0Alhl+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhh+hhh+%3B+hhl+lll+lhl,"Aries Dark Region JW-W c1-4
+https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+KH-V+c2-4&data=hlh+hhh+%3B+hhl+lll+lhl%0D%0Ahlh+lll+%3B+lll+llh+lhh%0D%0Alll+hll+lhl+%3B+lll+llh+lhh
+","Pleiades Sector MX-U d2-63
+https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+KH-V+c2-4&data=hhl+lhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lll+llh+%3B+hhl+lll+lhl
+","Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.
+",3,Yes. It has 1 cave-in by a(n exterior) door and 1 cave-in in the tunnels.,4,,
+10/12/2019 19:32:15,ByteSturm,HIP 14702,1 C,https://i.imgur.com/slQhK8a.png,Small Reposing Leviathans,2,N/A,No,,,,"Hippity hoppity,
+The aliens wants me off their property.",5,N/A,1,,
+10/12/2019 19:38:13,ByteSturm,Aries Dark Region VT-R b4-0,A 6,https://i.imgur.com/lhH7ltV.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Pleiades Sector MX-U d2-63 https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+VT-R+b4-0&data=hhl+lhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+%3B+hlh+lhh%0D%0Ahhl+lll+llh+%3B+hhl+lll+lhl,"HIP 14702
+https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+VT-R+b4-0&data=hhl+hhl+hhl+%3B+lll+llh+lhh%0D%0Ahlh+hll+%3B+lll+llh+lhh%0D%0Allh+hhl+%3B+llh+hlh
+","Pleiades Sector MX-U d2-54
+https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+VT-R+b4-0&data=hhl+lhh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+llh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Alhl+hhl+%3B+lhl+hhh
+","Another site down,
+Another off the list.
+Scores more to go,
+I'm starting to feel pissed.",5,Yes. It has 1 cave-in by a(n exterior) door.,4,,
+10/12/2019 19:50:50,ByteSturm,Aries Dark Region HR-W d1-34,2 A,https://i.imgur.com/zT9aWJm.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Aries Dark Region NN-T c3-12 https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+HR-W+d1-34&data=hlh+hhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hll+%3B+hll+lll+hlh%0D%0Ahlh+hhh+lhh+hll+%3B+hhl+lll+lhl+hhh,"Hyades Sector PC-V b2-3
+https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+HR-W+d1-34&data=lhl+hll+%3B+hll+lll+hlh%0D%0Alll+hhl+%3B+lll+llh+lhh%0D%0Ahhl+lll+hlh+hhl+%3B+hhl+lll+lhl+hhh
+","Aries Dark Region VT-R b4-0
+https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+HR-W+d1-34&data=hll+lll+%3B+hll+lll+hlh%0D%0Ahll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lll+lhl+lll+%3B+hhl+lll+lhl+hhh
+","Some miles driven,
+Some fuel spent,
+THE EMPEROR APPROVES,
+AND I SHALL MAKE THE XENOS REPENT!",5,Yes. It has 1 cave-in by a(n exterior) door and 1 cave-in in the tunnels.,4,,
+10/12/2019 19:52:00,ByteSturm,Pleiades Sector LN-T c3-11,9 A,https://i.imgur.com/yLBhAXW.png,No Leviathans,2,N/A,No,,,,"Hippity hoppity,
+The aliens wants me off their property.",3,N/A,0,,
+10/12/2019 20:08:24,ByteSturm,Pleiades Sector HH-V c2-6,2 A,https://i.imgur.com/kggyXOE.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Pleiades Sector JC-V d2-62 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+HH-V+c2-6&data=hll+hll+%3B+hll+lll+hlh%0D%0Ahhl+hlh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhh+lhh+hll+%3B+hhl+lll+lhl+hhh,"Pleiades Sector JC-V c2-9
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+HH-V+c2-6&data=lll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhl+%3B+hll+hhl+hhh%0D%0Alhh+hhh+lll+%3B+hll+lll+hlh
+","Pleiades Sector HR-W d1-57
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+HH-V+c2-6&data=llh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhl+%3B+hll+lll+hlh%0D%0Ahhl+hhh+hhh+lhl+%3B+lll+llh+lhh
+","Some miles driven,
+Some fuel spent,
+THE EMPEROR APPROVES,
+AND I SHALL MAKE THE XENOS REPENT!",3,Yes. It has 1 cave-in by a(n exterior) door.,4,,
+10/12/2019 20:10:40,ByteSturm,Pleiades Sector IH-V c2-3,1 B,https://i.imgur.com/P4Ye5jV.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,HIP 17694 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+IH-V+c2-3&data=hhl+%3B+hhl+lll+lhl%0D%0Ahhl+lhl+%3B+hll+lll+hlh%0D%0Alll+llh+hhl+%3B+lll+llh+lhh,"Delphi
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+IH-V+c2-3&data=hll+%3B+hhl+lhh+lhh%0D%0Ahhl+%3B+hlh+lhh%0D%0Ahll+lll+hhl+%3B+hll+lll+hlh
+","Result: The three spheres do not intersect!
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+IH-V+c2-3&data=llh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhh+hll+hll+%3B+hhl+lll+lhl+hhh
+","Experienced a hyperdiction between the barnacle site after this one and Pleiades Sector JS-T c3-17. I boosted away and the Thargoid pulsed me offline but showed no interest in chasing after me. Usually how these hyperdictions go.
+",3,Yes. It has a cave-in by a(n exterior) door.,4,,
+10/12/2019 20:11:50,ByteSturm,Pleiades Sector JS-T c3-17,1 A,https://i.imgur.com/T81fDK5.png,No Leviathans,2,N/A,No,,,,"Another site down,
+Another off the list.
+Scores more to go,
+I'm starting to feel pissed.",3,N/A,0,,
+10/12/2019 20:13:27,ByteSturm,HIP 14756,2 E,https://i.imgur.com/TvmDbnb.png,Small Reposing Leviathans,2,N/A,No,,,,"Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.",3,N/A,2,,
+10/12/2019 20:14:45,ByteSturm,Synuefai UZ-M d8-63,B 1 A,https://i.imgur.com/GDwZ40O.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Col 285 Sector GW-W d1-66 https://tool.canonn.tech/linkdecoder/?origin=Synuefai+UZ-M+d8-63&data=lhl+hll+%3B+hll+lll+hlh%0D%0Ahhl+hll+%3B+hll+hhl+hhh%0D%0Ahlh+hlh+hhl+hhl+%3B+hhl+lll+lhl+hhh,"Col 285 Sector BQ-X c1-10
+https://tool.canonn.tech/linkdecoder/?origin=Synuefai+UZ-M+d8-63&data=hlh+lhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Allh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hlh+hhh+hhl+%3B+hhl+lll+lhl+hhh
+","Col 285 Sector EB-X d1-39
+https://tool.canonn.tech/linkdecoder/?origin=Synuefai+UZ-M+d8-63&data=hll+%3B+hlh+lhh%0D%0Allh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhh+lll+hhl+%3B+lll+llh+lhh
+","Monotony sets in,
+My SRV feels inert.
+I scan another chamber
+As a Goid egg goes 'splurt'.",3,Yes. It has 1 cave-in by a(n exterior) door and 1 cave-in in the tunnels.,4,,
+10/12/2019 20:16:59,ByteSturm,Synuefai RK-Y c17-3,A 1 A,https://i.imgur.com/PrdlGrb.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,HIP 14756 https://tool.canonn.tech/linkdecoder/?origin=Synuefai+RK-Y+c17-3&data=hhl+llh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lhl+%3B+hll+lll+hlh%0D%0Ahll+hll+lll+%3B+hll+hhl+hhh,"HIP 16692
+https://tool.canonn.tech/linkdecoder/?origin=Synuefai+RK-Y+c17-3&data=hlh+lhh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hll+%3B+hhl+lhh+lhh%0D%0Ahlh+hhl+hll+%3B+hhl+lll+lhl
+","HIP 14969
+https://tool.canonn.tech/linkdecoder/?origin=Synuefai+RK-Y+c17-3&data=hhl+hlh+hhl+%3B+lll+llh+lhh%0D%0Ahhl+%3B+lhl+hhh%0D%0Ahlh+hlh+hhh+%3B+hhl+lll+lhl
+","Had a Thargoid visitor that came, pulsed, and left as I was boarding my landed ship with my SRV.
+",3,Yes. It has 1 cave-in by a(n exterior) door and 1 cave-in in the tunnels.,4,,
+10/12/2019 20:18:03,ByteSturm,Col 285 Sector BQ-X c1-10,1 A,https://i.imgur.com/ZhGC3Tf.png,No Leviathans,2,N/A,No,,,,"Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.",4,N/A,0,,
+10/12/2019 20:19:15,ByteSturm,Col 285 Sector EB-X d1-39,4 A,https://i.imgur.com/aFj9RLW.png,Small Reposing Leviathans,2,N/A,No,,,,"I've started a race
+To get through this place
+Before a random Goid
+Scatters my ship to the void.",3,N/A,1,,
+10/12/2019 20:20:36,ByteSturm,HIP 12967,1 A,https://i.imgur.com/9tQLlUA.png,Small Reposing Leviathans,2,N/A,No,,,,"Experienced another hyperdiction between here and Aries Dark Region AQ-Y d14. I had to boost multiple times before the Thargoid gave up this time.
+",4,N/A,2,,
+10/12/2019 20:23:01,ByteSturm,Aries Dark Region AQ-Y d14,4 A,https://i.imgur.com/5r7y2kt.png,No Leviathans,2,N/A,No,,,,"My engine's humming,
+My heartbeat's thrumming,
+The LZ's not clear,
+The Goids draw near!",4,N/A,0,,
+10/12/2019 20:24:04,ByteSturm,Col 285 Sector GW-W d1-66,A 3,https://i.imgur.com/1b34SAC.png,Small Reposing Leviathans,2,N/A,No,,,,"Monotony sets in,
+My SRV feels inert.
+I scan another chamber
+As a Goid egg goes 'splurt'.",3,N/A,1,,
+10/12/2019 20:26:46,ByteSturm,Aries Dark Region JS-T c3-1,1 A,https://i.imgur.com/e3pCvTX.png,Small Reposing Leviathans,2,N/A,No,,,,"I've come across
+A geological aberration,
+The center of which
+Hides a bright constellation.",5,N/A,2,,
+10/12/2019 20:28:26,ByteSturm,HIP 11981,A 5 A,https://i.imgur.com/BQcdCk3.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Col 285 Sector GW-W d1-66 https://tool.canonn.tech/linkdecoder/?origin=HIP+11981&data=lhl+hll+%3B+hll+lll+hlh%0D%0Ahll+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hlh+hhl+hhl+%3B+hhl+lll+lhl+hhh,"HIP 12967
+https://tool.canonn.tech/linkdecoder/?origin=HIP+11981&data=hlh+hll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahll+lhl+%3B+hhl+lll+lhl+hhh%0D%0Alll+hhl+%3B+llh+hlh
+","Aries Dark Region JS-T c3-1
+https://tool.canonn.tech/linkdecoder/?origin=HIP+11981&data=hlh+lhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lhl+%3B+lll+llh+lhh%0D%0Alhh+hll+lll+%3B+hll+lll+hlh
+","Flitting red eyes
+Flit through the night,
+Droning 'long
+The halls of this site.",3,Yes. It has 1 cave-in by a(n exterionr) door and 1 cave-in in the tunnels.,4,,
+10/12/2019 20:29:36,ByteSturm,HIP 12916,4 A,https://i.imgur.com/KbjY00u.png,Small Reposing Leviathans,2,N/A,No,,,,"Flying through the dark
+The sight before me is stark.
+Spires litter the ground
+Of the next site I have found.",3,N/A,1,,
+10/12/2019 20:30:53,ByteSturm,Aries Dark Region FW-W d1-59,A 5 A,https://i.imgur.com/pBj1jD3.png,Small Reposing Leviathans,2,N/A,No,,,,"I've started a race
+To get through this place
+Before a random Goid
+Scatters my ship to the void.",3,N/A,2,,
+10/12/2019 20:32:33,ByteSturm,Aries Dark Region FW-W d1-76,4 A,https://i.imgur.com/N1tsaLH.png,No Leviathans,2,N/A,No,,,,"Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.",5,N/A,0,,
+10/12/2019 20:34:18,ByteSturm,Aries Dark Region DB-X d1-64,4 A,https://i.imgur.com/sAL1zp4.png,Small Reposing Leviathans,2,N/A,No,,,,"Monotony sets in,
+My SRV feels inert.
+I scan another chamber
+As a Goid egg goes 'splurt'.",3,N/A,2,,
+10/12/2019 20:35:36,ByteSturm,Aries Dark Region DB-X d1-63,A 7 A,https://i.imgur.com/vosakQg.png,No Leviathans,2,N/A,No,,,,"Another site down,
+Another off the list.
+Scores more to go,
+I'm starting to feel pissed.",3,N/A,0,,
+10/12/2019 20:37:08,ByteSturm,Col 285 Sector RI-R b5-3,8 B,https://i.imgur.com/yndu0Pf.png,Small Reposing Leviathans,2,N/A,No,,,,"Some miles driven,
+Some fuel spent,
+THE EMPEROR APPROVES,
+AND I SHALL MAKE THE XENOS REPENT!",3,N/A,1,,
+10/12/2019 20:38:41,ByteSturm,HIP 16692,4 C,https://i.imgur.com/RX0qKwx.png,Small Reposing Leviathans,2,N/A,No,,,,"Another site down,
+Another off the list.
+Scores more to go,
+I'm starting to feel pissed.",5,N/A,1,,
+10/12/2019 20:39:38,ByteSturm,Hyades Sector DB-X c1-8,3 A,https://i.imgur.com/PKfmMJk.png,Small Reposing Leviathans,2,N/A,No,,,,"Hippity hoppity,
+The aliens wants me off their property.",3,N/A,1,,
+10/12/2019 20:41:03,ByteSturm,Hyades Sector DB-X c1-19,8 C,https://i.imgur.com/5JLtnxt.png,No Leviathans,2,N/A,No,,,,"Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.",3,N/A,0,,
+10/12/2019 20:44:26,ByteSturm,Hyades Sector ZU-Y c16,6 B,https://i.imgur.com/y2evdi8.png,Small Reposing Leviathans,2,N/A,No,,,,"Monotony sets in,
+My SRV feels inert.
+I scan another chamber
+As a Goid egg goes 'splurt'.",3,N/A,2,,
+10/12/2019 20:46:11,ByteSturm,Hyades Sector AQ-Y d93,2 B,https://i.imgur.com/Hi5G0ff.png,No Leviathans,2,N/A,No,,,,"My engine's humming,
+My heartbeat's thrumming,
+The LZ's not clear,
+The Goids draw near!",3,N/A,0,,
+10/12/2019 20:49:54,ByteSturm,Hyades Sector AQ-Y d81,AB 3 C,https://i.imgur.com/t7mzep8.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Etain https://tool.canonn.tech/linkdecoder/?origin=Hyades+Sector+AQ-Y+d81&data=llh+lhh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Alll+hll+%3B+hll+hhl+hhh%0D%0Ahlh+hlh+hll+%3B+hhl+lll+lhl,"Zavijah
+https://tool.canonn.tech/linkdecoder/?origin=Hyades+Sector+AQ-Y+d81&data=lll+hhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Alhl+hhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hlh+lhl+lll+%3B+hhl+lll+lhl+hhh
+",,"I drove one eve,
+Long hallowed halls,
+Saw an alien,
+And realized I had no balls.",5,Yes. It has 1 cave-in by a(n exterior) door and 1 cave-in in the tunnels.,4,,
+10/12/2019 20:54:01,ByteSturm,Pleiades Sector OY-R c4-19,A 3 A,https://i.imgur.com/dkQheqp.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Col 285 Sector RI-R b5-3 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+OY-R+c4-19&data=hlh+hll+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lhl+%3B+hll+hhl+hhh%0D%0Ahll+hll+lll+%3B+hll+hhl+hhh,"Hyades Sector AQ-Y d81
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+OY-R+c4-19&data=hll+hll+%3B+hll+hhl+hhh%0D%0Ahll+%3B+hhl+lhh+lhh%0D%0Alhl+hll+%3B+lhl+hhh
+","Pleiades Sector VK-N b7-0
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+OY-R+c4-19&data=hhl+hhl+%3B+hhl+lhh+lhh%0D%0Ahhl+hll+%3B+lll+llh+lhh%0D%0Ahlh+hhh+hll+hhl+%3B+hhl+lll+lhl+hhh
+","Some miles driven,
+Some fuel spent,
+THE EMPEROR APPROVES,
+AND I SHALL MAKE THE XENOS REPENT!",4,Yes. It has 1 cave-in by a(n exterior) door and 2 cave-ins in the tunnels.,4,,
+10/12/2019 20:55:03,ByteSturm,Pleiades Sector VK-N b7-0,C 1,https://i.imgur.com/AMRcCva.png,No Leviathans,2,N/A,No,,,,"Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.
+",3,N/A,0,,
+10/12/2019 21:00:09,ByteSturm,Pleiades Sector FW-W d1-37,2 A,https://i.imgur.com/aK9nse5.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,HIP 20964 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+FW-W+d1-37&data=lhh+lll+%3B+hll+lll+hlh%0D%0Ahhl+hhh+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhh+hhh+lll+%3B+lll+llh+lhh,"HIP 14908
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+FW-W+d1-37&data=hlh+hhl+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhl+%3B+hhl+lhh+lhh%0D%0Alhh+hhl+hll+%3B+hll+lll+hlh
+","HIP 18909
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+FW-W+d1-37&data=hhl+hhh+lll+%3B+lll+llh+lhh%0D%0Alhl+hll+%3B+lll+llh+lhh%0D%0Ahhl+lll+lll+%3B+hhl+lll+lhl
+","I've started a race
+To get through this place
+Before a random Goid
+Scatters my ship to the void.",3,Yes. It has 1 cave-in by a(n exterior) door.,4,,
+11/12/2019 02:49:18,ByteSturm,Pleiades Sector LS-T c3-2,5 A,https://i.imgur.com/TvwG14f.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Hyades Sector DB-X c1-19 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+LS-T+c3-2&data=hll+lll+%3B+hll+hhl+hhh%0D%0Ahlh+lll+%3B+hll+lll+hlh%0D%0Alhh+hhl+lll+%3B+hll+lll+hlh,"Pleiades Sector GW-W d1-54
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+LS-T+c3-2&data=hhl+hll+%3B+hhl+lhh+lhh%0D%0Alll+%3B+hll+hhl+hhh%0D%0Alll+lll+lll+%3B+lll+llh+lhh
+","Pleiades Sector FW-W d1-37
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+LS-T+c3-2&data=hhl+lhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahll+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhh+hhl+lll+%3B+lll+llh+lhh
+","Hippity hoppity,
+The aliens wants me off their property.",4,Yes. It has 1 cave-in by a(n exterior) door.,4,,
+11/12/2019 02:50:49,ByteSturm,Pleiades Sector MX-U d2-54,4 E,https://i.imgur.com/2UcBHZM.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,HIP 16440 https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+MX-U+d2-54&data=lhl+hll+%3B+lll+llh+lhh%0D%0Alll+%3B+hll+lll+hlh%0D%0Ahhl+hhh+hhh+lll+%3B+lll+llh+lhh,"Result: The three spheres do not intersect!
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+MX-U+d2-54&data=hhl+hhl+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahll+%3B+hhl+lll+lhl%0D%0Ahhl+lhh+lhl+%3B+hhl+lhh+lhh
+","Aries Dark Region GW-W d1-48
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+MX-U+d2-54&data=hhl+llh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahll+%3B+hhl+lll+lhl%0D%0Ahhl+lll+llh+lhl+%3B+hhl+lll+lhl+hhh
+","Monotony sets in,
+My SRV feels inert.
+I scan another chamber
+As a Goid egg goes 'splurt'.",5,Yes. It has 2 cave-ins in the tunnels and 1 by a(n exterior) door.,4,,
+11/12/2019 02:52:36,ByteSturm,HIP 17837,A 4 B,https://i.imgur.com/th6Go2Q.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Result: The three spheres do not intersect! https://tool.canonn.tech/linkdecoder/?origin=HIP+17837&data=hhl+llh+lll+%3B+hll+lll+hlh%0D%0Ahll+hhl+hll+%3B+lll+llh+lhh%0D%0Alhl+hhh+lll+%3B+hll+lll+hlh,"Nogambe
+https://tool.canonn.tech/linkdecoder/?origin=HIP+17837&data=hll+hlh+hll+%3B+lll+llh+lhh%0D%0Alhl+hll+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahll+hlh+hll+%3B+hll+hhl+hhh
+",,"Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.",4,Yes. It has 1 cave-in by a(n exterior) door.,4,,
+11/12/2019 02:55:58,ByteSturm,HIP 17044,1 B,https://i.imgur.com/BEE1GEL.png,Large upright leviathans,4,"Yes, 4 around the central chamber.",Yes,HIP 11981 https://tool.canonn.tech/linkdecoder/?origin=HIP+17044&data=hlh+hll+%3B+hhl+lll+lhl%0D%0Ahhl+lhl+hll+%3B+hhl+lll+lhl+hhh%0D%0Ahll+lhh+hll+%3B+hll+hhl+hhh,"HIP 17837
+https://tool.canonn.tech/linkdecoder/?origin=HIP+17044&data=hhl+hhl+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lhl+%3B+lll+llh+lhh%0D%0Ahhl+lhh+lhl+%3B+hhl+lhh+lhh
+","Aries Dark Region KH-V c2-4
+https://tool.canonn.tech/linkdecoder/?origin=HIP+17044&data=lll+lll+%3B+lll+llh+lhh%0D%0Ahhl+hlh+lll+%3B+hhl+lll+lhl+hhh%0D%0Alll+llh+hll+%3B+lll+llh+lhh
+","I've started a race
+To get through this place
+Before a random Goid
+Scatters my ship to the void.",3,No.,4,,
+11/12/2019 02:58:58,ByteSturm,HIP 16440,1 E,https://i.imgur.com/YubvAVw.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 4 around the central chamber.",Yes,Result: The three spheres do not intersect! https://tool.canonn.tech/linkdecoder/?origin=HIP+16440&data=llh+lhl+%3B+hll+lll+hlh%0D%0Ahlh+hhh+%3B+hhl+lll+lhl%0D%0Ahhl+hhh+llh+lll+%3B+lll+llh+lhh,"Nauni
+https://tool.canonn.tech/linkdecoder/?origin=HIP+16440&data=hlh+lll+%3B+hhl+lhh+lhh%0D%0Alhh+lhl+%3B+hll+lll+hlh%0D%0Alhh+hhl+lll+%3B+hll+lll+hlh
+",,"I've come across
+A geological aberration,
+The center of which
+Hides a bright constellation.",5,No.,4,,
+11/12/2019 03:13:44,ByteSturm,Aries Dark Region IH-V c2-9,6 B,https://i.imgur.com/HnxgDSR.png,Small Reposing Leviathans,4,N/A,No,,,,"Flitting red eyes
+Flit through the night,
+Droning 'long
+The halls of this site.",5,N/A,2,,
+11/12/2019 03:15:41,ByteSturm,HIP 11154,B 9 B,https://i.imgur.com/Ih0ns8e.png,Small Reposing Leviathans,2,N/A,No,,,,"Flying through the dark
+The sight before me is stark.
+Spires litter the ground
+Of the next site I have found.",5,N/A,2,,
+11/12/2019 03:21:35,ByteSturm,Aries Dark Region OI-T c3-8,1 A,https://i.imgur.com/hdPL5oG.png,No Leviathans,2,N/A,No,,,,"My engine's humming,
+My heartbeat's thrumming,
+The LZ's not clear,
+The Goids draw near!",3,N/A,0,,
+11/12/2019 03:23:10,ByteSturm,Aries Dark Region MX-U c2-10,1 C,https://i.imgur.com/tg9Jlhl.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,HIP 15329 https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+MX-U+c2-10&data=lll+hhl+%3B+hll+lll+hlh%0D%0Ahll+lhh+%3B+hhl+lll+lhl%0D%0Ahll+lhh+hll+%3B+hll+hhl+hhh,"Nogambe
+https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+MX-U+c2-10&data=hll+hlh+hll+%3B+lll+llh+lhh%0D%0Ahll+hlh+hll+%3B+lll+llh+lhh%0D%0Ahll+hlh+hll+%3B+hll+hhl+hhh
+",,"I drove one eve,
+Long hallowed halls,
+Saw an alien,
+And realized I had no balls.",3,Yes. It has 1 cave-in by a(n exterior) door.,4,,
+11/12/2019 03:25:28,ByteSturm,Aries Dark Region JC-V c2-6,A 4 A,https://i.imgur.com/myBSCJC.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Aries Dark Region PY-R b4-1 https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+JC-V+c2-6&data=hlh+hhh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhl+%3B+hll+lll+hlh%0D%0Ahll+hll+hll+%3B+hll+hhl+hhh,"Aries Dark Region IH-V c2-9
+https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+JC-V+c2-6&data=hhl+lhl+%3B+hhl+lhh+lhh%0D%0Ahhl+hlh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhh+llh+lhl+%3B+hhl+lll+lhl+hhh
+","Aries Dark Region HR-W d1-53
+https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+JC-V+c2-6&data=hlh+hhl+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahll+%3B+hhl+lll+lhl%0D%0Alhh+hlh+hhl+%3B+hll+lll+hlh
+","Some miles driven,
+Some fuel spent,
+THE EMPEROR APPROVES,
+AND I SHALL MAKE THE XENOS REPENT!",3,Yes. It has 1 cave-in in the tunnels and 1 cave-in by a(n exterior) door.,4,,
+11/12/2019 03:27:17,ByteSturm,Aries Dark Region HR-W d1-44,B 2 A,https://i.imgur.com/wYy4eWW.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Aries Dark Region JC-V c2-6 https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+HR-W+d1-44&data=hhl+hlh+hll+%3B+lll+llh+lhh%0D%0Alhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lhl+lll+%3B+hhl+lhh+lhh,"Aries Dark Region FW-W d1-76
+https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+HR-W+d1-44&data=hlh+lhl+%3B+hhl+lll+lhl%0D%0Ahhl+hlh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Alhh+hll+hll+%3B+hll+lll+hlh
+","HIP 12916
+https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+HR-W+d1-44&data=lhh+hhl+%3B+hll+lll+hlh%0D%0Ahhl+lhl+%3B+hll+hhl+hhh%0D%0Ahlh+hhl+hlh+%3B+hhl+lll+lhl
+","Another site down,
+Another off the list.
+Scores more to go,
+I'm starting to feel pissed.",3,Yes. It has 1 cave-in by a(n exterior) door.,4,,
+11/12/2019 03:29:05,ByteSturm,Aries Dark Region PY-R b4-1,A 4 A,https://i.imgur.com/JKtPY9H.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Conn https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+PY-R+b4-1&data=hhl+lhh+lhl+%3B+hll+lll+hlh%0D%0Alhl+llh+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hlh+lll+%3B+hhl+lll+lhl,"Gertrud
+https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+PY-R+b4-1&data=hlh+hll+%3B+llh+hlh%0D%0Alhl+lhl+hhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hlh+hlh+hhl+%3B+hhl+lll+lhl+hhh
+",,"Hippity hoppity,
+The aliens wants me off their property.",3,Yes. It has 1 cave-in in the tunnels and 1 cave-in by a(n exterior) door.,4,,
+11/12/2019 03:31:10,ByteSturm,Aries Dark Region HR-W d1-53,7 A,https://i.imgur.com/bcZGxET.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Aries Dark Region JC-V c2-4 https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+HR-W+d1-53&data=hhl+hlh+hll+%3B+lll+llh+lhh%0D%0Ahll+%3B+hhl+lll+lhl%0D%0Ahhl+lhl+lll+%3B+hhl+lhh+lhh,"Aries Dark Region DB-X d1-63
+https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+HR-W+d1-53&data=hll+hll+%3B+hll+hhl+hhh%0D%0Ahlh+hhl+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahll+hll+lhl+%3B+hll+hhl+hhh
+","Result: The three spheres do not intersect!
+https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+HR-W+d1-53&data=hlh+hhh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhl+%3B+lll+llh+lhh%0D%0Ahll+hll+hll+%3B+hll+hhl+hhh
+","Monotony sets in,
+My SRV feels inert.
+I scan another chamber
+As a Goid egg goes 'splurt'.",5,Yes. It has 1 cave-in in the tunnels and 1 cave-in by a(n exterior) door.,4,,
+11/12/2019 03:33:02,ByteSturm,Aries Dark Region HR-W d1-54,2 C A,https://i.imgur.com/98f6DZl.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Aries Dark Region MX-U c2-8 https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+HR-W+d1-54&data=hlh+lhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+lhl+%3B+lll+llh+lhh%0D%0Alhh+hhl+lll+%3B+hll+lll+hlh,"Aries Dark Region JC-V c2-6
+https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+HR-W+d1-54&data=hhl+hlh+hll+%3B+lll+llh+lhh%0D%0Ahhl+lhl+%3B+lll+llh+lhh%0D%0Ahhl+lhl+lll+%3B+hhl+lhh+lhh
+","Aries Dark Region HR-W d1-53
+https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+HR-W+d1-54&data=hlh+hhl+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+%3B+llh+hlh%0D%0Alhh+hlh+hhl+%3B+hll+lll+hlh
+","I've started a race
+To get through this place
+Before a random Goid
+Scatters my ship to the void.",5,Yes. It has 1 cave-in by a(n exterior) door.,4,,
+11/12/2019 03:35:31,ByteSturm,Aries Dark Region MX-U c2-8,A 7 A,https://i.imgur.com/T0mpfQy.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Hermitage https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+MX-U+c2-8&data=hll+lhh+hll+%3B+lll+llh+lhh%0D%0Alll+hhl+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhl+lhl+hll+%3B+lll+llh+lhh,"HIP 9141
+https://tool.canonn.tech/linkdecoder/?origin=Aries+Dark+Region+MX-U+c2-8&data=hhl+hhl+hhl+%3B+hll+hhl+hhh%0D%0Ahlh+lhl+hll+%3B+lll+llh+lhh%0D%0Ahhl+hhh+lhl+lll+%3B+lll+llh+lhh
+",,"Since I started this trip
+It's been over a year.
+Now checking off my list
+Is all I hold dear.",3,Yes. It has 1 cave-in in the tunnels and 1 cave-in by a(n exterior) door.,4,,
+11/12/2019 03:38:04,ByteSturm,HIP 11700,1 A,https://i.imgur.com/D7j6HQE.png,"Large upright leviathans, Small Reposing Leviathans",4,"Yes, 1 in the tunnels.",Yes,Aries Dark Region HR-W d1-54 https://tool.canonn.tech/linkdecoder/?origin=HIP+11700&data=hll+%3B+hlh+lhh%0D%0Allh+lhl+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhl+hhh+hhl+%3B+hhl+lll+lhl+hhh,"HIP 11457
+https://tool.canonn.tech/linkdecoder/?origin=HIP+11700&data=hll+%3B+hlh+lhh%0D%0Alhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahlh+hhh+lhl+hll+%3B+hhl+lll+lhl+hhh
+","Result: The three spheres do not intersect!
+https://tool.canonn.tech/linkdecoder/?origin=HIP+11700&data=hlh+lhh+lll+%3B+hhl+lll+lhl+hhh%0D%0Ahhl+hhl+%3B+hll+lll+hlh%0D%0Alhh+hhl+lll+%3B+hll+lll+hlh
+","Monotony sets in,
+My SRV feels inert.
+I scan another chamber
+As a Goid egg goes 'splurt'.",5,Yes. It has 1 cave-in by a(n exterior) door.,4,,
+11/12/2019 03:39:28,ByteSturm,HIP 11457,1 C,https://i.imgur.com/Vli1iIS.png,No Leviathans,2,N/A,No,,,,"I've come across
+A geological aberration,
+The center of which
+Hides a bright constellation.",3,N/A,0,,
+11/12/2019 03:40:30,ByteSturm,HIP 14908,A 2 A,https://i.imgur.com/gzpWUCf.png,"Large upright leviathans, Small Reposing Leviathans",2,N/A,No,,,,"Flitting red eyes
+Flit through the night,
+Droning 'long
+The halls of this site.",3,N/A,2,,
+11/12/2019 03:41:47,ByteSturm,Aries Dark Region MS-T c3-15,1 A,https://i.imgur.com/ctS5KtP.png,Small Reposing Leviathans,2,N/A,No,,,,"Flying through the dark
+The sight before me is stark.
+Spires litter the ground
+Of the next site I have found.",3,N/A,2,,
+11/12/2019 03:42:39,ByteSturm,HIP 15134,3 B,https://i.imgur.com/4CqoT9T.png,Small Reposing Leviathans,2,N/A,No,,,,"My engine's humming,
+My heartbeat's thrumming,
+The LZ's not clear,
+The Goids draw near!",3,N/A,2,,
+11/12/2019 03:43:47,ByteSturm,Aries Dark Region NN-T c3-12,1 D,https://i.imgur.com/HeAKoUh.png,Small Reposing Leviathans,2,N/A,No,,,,"Void shrouded by darkness,
+Nothing survives, nothing remains.
+Suddenly LIGHT, COLOR, PIERCING, PARADING!
+LAUGHING AT THE DARKNESS!
+A single ship, it breaks apart.
+Now its twin shines blue.
+They're speaking but one's listening.
+The twin cries out, yelling, screaming voices.
+Silence, persisting, pervading.
+The ship sputters closer, grabs its sibling.
+Engines roar, a course is plotted,
+The ship departs.
+Void shrouded by darkness,
+Nothing survives, nothing remains.",3,N/A,1,,
+11/12/2019 03:45:57,ByteSturm,Pleiades Sector OD-S b4-1,2 A,https://i.imgur.com/RSp0swm.png,"Large upright leviathans, Small Reposing Leviathans",4,No.,Yes,Nu-1 Columbae https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+OD-S+b4-1&data=hlh+hll+%3B+llh+hlh%0D%0Ahhl+hhl+lll+%3B+hll+hhl+hhh%0D%0Ahlh+hhh+hll+lhl+%3B+hhl+lll+lhl+hhh,"Magec
+https://tool.canonn.tech/linkdecoder/?origin=Pleiades+Sector+OD-S+b4-1&data=lll+hlh+%3B+hhl+lll+lhl%0D%0Ahlh+hll+%3B+lhl+hhh%0D%0Alhl+%3B+lhh
+",,"Hippity hoppity,
+The aliens wants me off their property.",3,Yes. It has 1 cave-in in the tunnels and 1 cave-in by a(n exterior) door.,4,,
+11/12/2019 04:06:21,ByteSturm,Hind Sector GR-W d1-13,8 H,https://i.imgur.com/goJhVSW.png,,2,N/A,No,,,,Unsure of how many Leviathans because I hadn't updated how I record my findings since the beginning of this expedition and had taken a screenshot that was way too dark. No other way to tell.,1,N/A,2,,
+11/12/2019 04:08:25,ByteSturm,Pleiades Sector OS-U c2-7,4 A,https://i.imgur.com/moC0a1Z.jpg,,2,N/A,No,,,,Unsure of how many Leviathans. My input under 'DrunkEng1n33r' at the beginning of this expedition states that there are none.,1,N/A,0,,
+11/12/2019 04:10:33,ByteSturm,Mel 22 Sector AX-A b14-1,1 B,https://i.imgur.com/MYwCeTh.jpg,No Leviathans,2,N/A,No,,,,My last entry for this expedition. !!!!!!!!!!!!!!!!!!!!!!!111!!!!!!!1!!!!!!!!!!!!!!!!!!!!!!!!!!! I'm going to go freak out on Discord now. o7,5,N/A,0,,
+, ,,,,,,,,,,,,,,,,
\ No newline at end of file
diff --git a/data/csvCache/UIA Vector Survey (Responses) - Responses.csv b/data/csvCache/UIA Vector Survey (Responses) - Responses.csv
new file mode 100644
index 0000000..c4893b6
--- /dev/null
+++ b/data/csvCache/UIA Vector Survey (Responses) - Responses.csv
@@ -0,0 +1,41 @@
+Timestamp,Commander Name,Date and Time,Targetted System,Current System,Screenshot,Accuracy,Permit Lock
+03/09/2022 13:04:50,Martind Forlon,03/09/2022 11:41:00,Oochorrs CS-F c13-0,Oochorrs DS-F c13-0,https://cdn.discordapp.com/attachments/344094711339941890/1015561780770123796/From.jpg,5,
+03/09/2022 13:06:16,Martind Forlon,03/09/2022 11:41:00,Oochorrs UF-J c11-0,Oochorrs DS-F c13-0,https://cdn.discordapp.com/attachments/344094711339941890/1015561781017575444/To.jpg,5,
+03/09/2022 18:56:45,CodexNecro81,31/08/2022 20:28:00,Outotz AL-N d7-1,Oochorrs YV-I c11-0,https://cdn.discordapp.com/attachments/1015411592164286586/1015680238669271173/unknown.png,2,
+03/09/2022 18:58:20,CodexNecro81,31/08/2022 23:20:00,Outotz CW-L d8-0,Oochorrs YV-I c11-0,https://cdn.discordapp.com/attachments/1015411592164286586/1015680542290751498/unknown.png,1,
+03/09/2022 19:35:31,aran ilyaris,03/09/2022 18:31:00,Oochorrs GL-O d6-0,Oochorrs YL-H c12-0,https://cdn.discordapp.com/attachments/1015411592164286586/1015691388421144586/20220903203151_1.jpg,3,
+03/09/2022 20:25:18,CodexNecro81,01/09/2022 22:26:00,HD 267704,Oochorrs BH-H c12-0,https://cdn.discordapp.com/attachments/344094711339941890/1015026951192326175/unknown.png,1,
+03/09/2022 20:26:38,aran ilyaris,03/09/2022 19:20:00,Outotz CQ-O c6-0,Oochorrs FN-F c13-0,https://cdn.discordapp.com/attachments/805203782186172417/1015704288368013415/20220903212028_1.jpg,2,
+03/09/2022 21:21:54,aran ilyaris,03/09/2022 20:18:00,NGC 2264 Sector TU-W a60-0,Oochorrs NI-R d5-0,https://cdn.discordapp.com/attachments/805203782186172417/1015718225226498078/20220903221829_1.jpg,1,yes
+03/09/2022 22:23:27,aran ilyaris,03/09/2022 21:20:00,NGC 2264 Sector XL-L d8-0,Oochorrs NI-R d5-1,https://cdn.discordapp.com/attachments/805203782186172417/1015733737381703770/20220903232004_1.jpg,2,yes
+03/09/2022 22:31:16,Daest,31/08/2022 14:17:00,Outotz KB-G B39-0,Oochorrs WP-K C10-0,https://cdn.discordapp.com/attachments/344094711339941890/1014539394021072996/unknown.png,2,
+03/09/2022 22:33:24,Daest,31/08/2022 15:18:00,Oochorrs QJ-E B3-0,Oochorrs AM-H C12-0,https://cdn.discordapp.com/attachments/344094711339941890/1014554765272817744/unknown.png,1,
+03/09/2022 22:37:15,Daest,31/08/2022 22:16:00,NGC 1746 Sector YG-K C9-0,Oochorrs BB-J C11-0,https://cdn.discordapp.com/attachments/344094711339941890/1014661510448025601/unknown.png,1,
+03/09/2022 22:39:55,Daest,02/09/2022 23:17:00,Oochoxt YR-F B16-0,Oochorrs XL-H C12-0,https://cdn.discordapp.com/attachments/344094711339941890/1015218984305565706/unknown.png,1,
+03/09/2022 22:40:55,Daest,02/09/2022 23:40:00,Oochoxt PJ-C B18-0,Oochorrs XL-H C12-0,https://cdn.discordapp.com/attachments/344094711339941890/1015406350890713098/unknown.png,1,
+03/09/2022 22:41:41,Daest,02/09/2022 23:53:00,Oochorrs JX-Z C1-0,Oochorrs DS-F C13-0,https://cdn.discordapp.com/attachments/344094711339941890/1015409353349660812/unknown.png,2,
+03/09/2022 22:46:38,Daest,31/08/2022 23:23:00,Flame Sector CV-X B1-0,Oochorrs AV-W D2-0,https://cdn.discordapp.com/attachments/490250707153453056/1014676938293514390/unknown.png,1,
+03/09/2022 22:47:40,Daest,31/08/2022 23:46:00,NGC 2232 Sector QP-F B11-0,Oochorrs MM-A C2-0,https://cdn.discordapp.com/attachments/490250707153453056/1014682910726574191/unknown.png,1,
+04/09/2022 02:47:00,CodexNecro81,04/09/2022 01:45:00,Outotch SR-Z c14-0,Oochorrs AH-H c12-0,https://cdn.discordapp.com/attachments/1015411592164286586/1015799691574775819/unknown.png,2,
+04/09/2022 03:01:32,CodexNecro81,04/09/2022 01:59:00,Oochoxt VO-H d10-0,Oochorrs KN-R d5-1,https://cdn.discordapp.com/attachments/1015411592164286586/1015803447272030368/unknown.png,2,
+04/09/2022 07:38:40,aran ilyaris,04/09/2022 06:31:00,Col 97 Sector FB-L a38-0,Oochorrs AH-H c12-0,https://cdn.discordapp.com/attachments/805203782186172417/1015873410431197184/20220904083127_1.jpg,1,yes
+04/09/2022 19:14:00,CodexNecro81,04/09/2022 18:08:00,Outotz IM-K c22-2,Oochorrs AH-H c12-0,https://cdn.discordapp.com/attachments/1015411592164286586/1016047880403951677/Elite_Dangerous_Screenshot_2022.09.04_-_19.07.35.66.png,2,
+04/09/2022 19:15:49,CodexNecro81,04/09/2022 17:59:00,Oochoxt UC-O c21-0,Oochorrs KN-R d5-1,https://cdn.discordapp.com/attachments/1015411592164286586/1016047881192484945/Elite_Dangerous_Screenshot_2022.09.04_-_18.59.03.13.png,2,
+05/09/2022 13:57:57,CodexNecro81,05/08/2022 12:50:00,Flyua Dryoae OO-J b12-0,Oochorrs KN-R d5-2,https://cdn.discordapp.com/attachments/986218504116137995/1016330890080702514/unknown.png,1,
+05/09/2022 14:07:41,CodexNecro81,05/09/2022 13:04:00,Oochorrs PT-P d6-0,Oochorrs MY-P d6-6,https://cdn.discordapp.com/attachments/986218504116137995/1016333407317401640/Elite_Dangerous_Screenshot_2022.09.05_-_14.03.36.22.png,2,
+05/09/2022 16:46:21,CodexNecro81,05/09/2022 15:33:00,Outotch OU-N c7-0,Oochorrs OT-P d6-3,https://cdn.discordapp.com/attachments/986218504116137995/1016371095680585779/unknown.png,2,
+05/09/2022 16:47:40,CodexNecro81,05/09/2022 15:42:00,Flyua Dryoae WF-O d6-0,Oochorrs IS-R d5-1,https://cdn.discordapp.com/attachments/986218504116137995/1016372986414120980/Elite_Dangerous_Screenshot_2022.09.05_-_16.41.30.32.png,1,
+05/09/2022 18:37:09,CodexNecro81,05/09/2022 17:13:00,Oochorrs LP-L c9-0,Oochorrs PT-P d6-2,https://cdn.discordapp.com/attachments/986218504116137995/1016401152994181190/Elite_Dangerous_Screenshot_2022.09.05_-_18.13.15.14.png,2,
+05/09/2022 18:38:09,CodexNecro81,05/09/2022 17:24:00,Oochorrs KZ-B c28-0,Oochorrs IS-R d5-1,https://cdn.discordapp.com/attachments/986218504116137995/1016401240231526491/Elite_Dangerous_Screenshot_2022.09.05_-_18.23.42.65.png,1,
+05/09/2022 21:01:12,CodexNecro81,05/09/2022 19:58:00,Synuefe JP-G c11-0,Oochorrs IS-R d5-1,https://cdn.discordapp.com/attachments/986218504116137995/1016437437569957908/Elite_Dangerous_Screenshot_2022.09.05_-_20.57.54.44.png,1,
+05/09/2022 21:13:53,CodexNecro81,05/09/2022 20:12:00,Oochost QH-U d3-1,Oochorrs PT-P d6-2,https://cdn.discordapp.com/attachments/986218504116137995/1016440971547914311/Elite_Dangerous_Screenshot_2022.09.05_-_21.11.37.64.png,1,
+06/09/2022 00:08:56,CodexNecro81,05/09/2022 22:55:00,Flyua Dryoae RW-R c18-0,Oochorrs IS-R d5-1,https://cdn.discordapp.com/attachments/986218504116137995/1016484638820139129/Elite_Dangerous_Screenshot_2022.09.05_-_23.55.26.12.png,2,
+06/09/2022 00:10:02,CodexNecro81,05/09/2022 23:05:00,Oochorrs QG-I c11-0,Oochorrs PT-P d6-2,https://cdn.discordapp.com/attachments/986218504116137995/1016484833054171246/Elite_Dangerous_Screenshot_2022.09.06_-_00.05.13.69.png,2,
+06/09/2022 11:48:11,CodexNecro81,06/09/2022 10:31:00,Tr 10 Sector PX-U b2-4,Oochorrs WL-H c12-0,https://cdn.discordapp.com/attachments/986218504116137995/1016660739345371177/Elite_Dangerous_Screenshot_2022.09.06_-_11.30.28.95.png,1,
+06/09/2022 11:49:01,CodexNecro81,06/09/2022 10:45:00,Outotch AX-R c5-0,Oochorrs GT-D c14-0,https://cdn.discordapp.com/attachments/986218504116137995/1016660827094401075/Elite_Dangerous_Screenshot_2022.09.06_-_11.45.04.55.png,2,
+06/09/2022 12:42:19,Verbotron,06/09/2022 08:27:00,Oochorrs SZ-N d7-2,Oochorrs MY-P d6-5,https://discord.com/channels/756485455456895068/1015515038422933514/1016674487246270504,2,
+06/09/2022 13:12:22,Verbotron,06/09/2022 12:09:00,Oochorrs BS-F c13-0,HD 38291,https://discord.com/channels/756485455456895068/1015515038422933514/1016681587066286081,1,
+06/09/2022 13:46:33,Verbotron,06/09/2022 12:43:00,Oochorrs BS-F c13-0,HD 38291,https://discord.com/channels/146714487695605760/296717350575800320/1016690433486766201,1,
+06/09/2022 22:23:41,CodexNecro81,06/09/2022 21:09:00,Col 132 Sector BE-B a61-0,Oochorrs WL-H c12-0,https://cdn.discordapp.com/attachments/986218504116137995/1016820456269025370/Elite_Dangerous_Screenshot_2022.09.06_-_22.08.59.43.png,2,
+06/09/2022 22:24:59,CodexNecro81,06/09/2022 21:19:00,Outotch GN-S d4-0,Oochorrs GT-D c14-0,https://cdn.discordapp.com/attachments/986218504116137995/1016820745483063369/Elite_Dangerous_Screenshot_2022.09.06_-_22.18.44.48.png,2,
+07/09/2022 01:52:58,CodexNecro81,07/09/2022 00:47:00,Cone Sector FL-Y c1,Oochorrs KZ-B c15-0,https://cdn.discordapp.com/attachments/986218504116137995/1016873454701662298/Elite_Dangerous_Screenshot_2022.09.07_-_01.46.52.12.png,1,
\ No newline at end of file
diff --git a/data/csvCache/adamastor.csv b/data/csvCache/adamastor.csv
new file mode 100644
index 0000000..8c05d56
--- /dev/null
+++ b/data/csvCache/adamastor.csv
@@ -0,0 +1,52 @@
+name,pos_x,pos_y,pos_z,infos
+Synuefe XE-Y c17-7,218.78125 , -102.4375 , -279.34375,
Adamastor Log: 29/10/3111
Entering system with a single K class star and 11 bodies.
Heading for third body.
Arrived at listening post.
Updating route to rendezvous with planetary survey team.
Listening Post found in Synuefe XE-Y C17-7. The location matches the description found in the flight of the Adamastor, so it is believed that the Listening Post is related. It has not been decoded, so we do not know what the message is, or if indeed it is decodable. The Listening Post was found by following a path from Chukchan through HIP 39748 and HIP 33386 and travelling for around 370 Ly then looking for a K class star with 11 bodies. It is believed that the Listening Post will probably point to Site 23B.
Message:
DATA DOWNLOAD
8BFGTY4PLU67-RTYO06.45:GN63-74PHGJI E67-:F563-21-574.9 ER34.6-DER8+WEST U.5 -RTG10 RTH8-4 6T.WR4564-21 +G134.2 RT55.4 GDW THE42.1LY 764.2Y-45TG4.BTJ-Y.6ORT437.1D341-67.Y5DS 243 45TY-3234 60RT437.10341-87.Y5DS 243 45TY-3234
Note that the message has been found to be slightly different in various localisations – notably in French the ‘WEST’ text is ‘OUEST’. It is not known if this is deliberate, or a mistake.
+HIP 39748, 173.40625,1.59375,-63.1875,Adamastor Original Waypoint
+HIP 33386, 195.625, -36.65625 , -141.6875,Adamastor Original Waypoint
+Musca Dark Region PJ-P B6-1, 432.625 , 2.53125 , 288.6875, 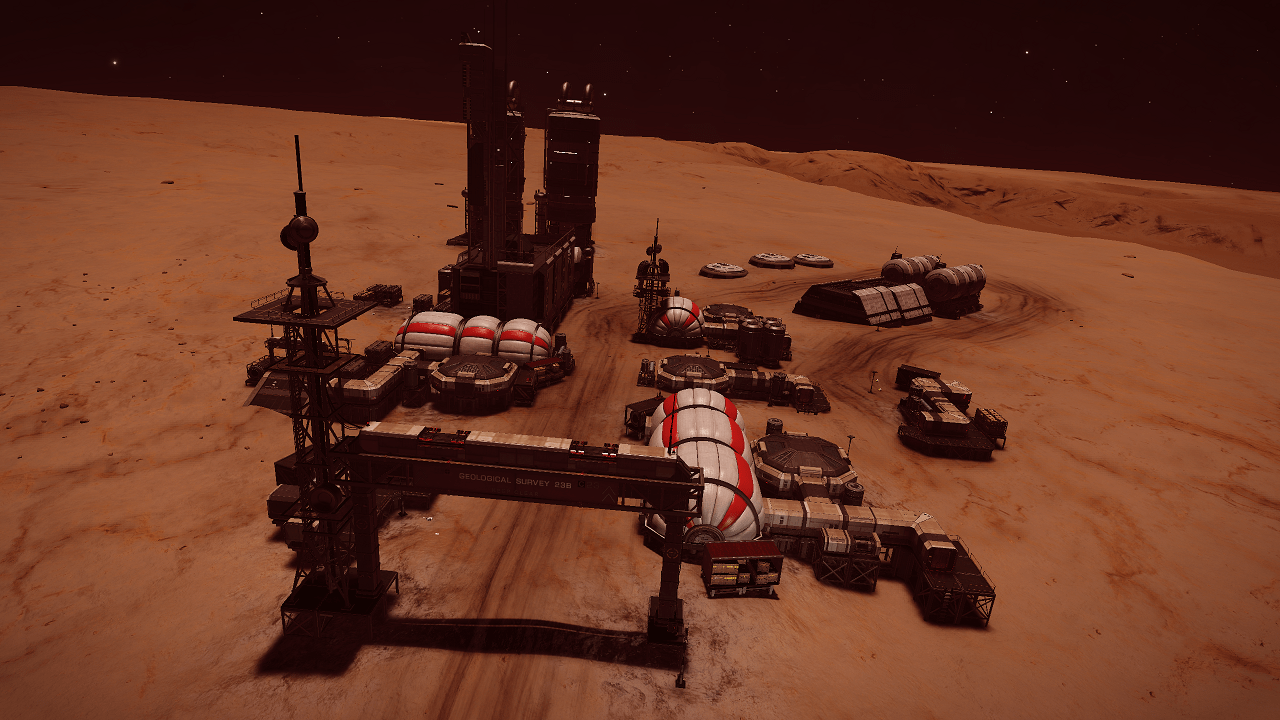
This abandoned settlement on Musca Dark Region PJ-P B6-1 3 in the Coalsack contains audio messages related to an expedition led by Penelope Carver funded by Azimuth Biochemicals and which was recovered by the Adamastor megaship. In 3111 the survey team found Thargoid Barnacles and a crashed ship (on the same planet). This was an early contact with Thargoid technology, probably leading to the recovery of the Thargoid Probe or Sensor.
There are four settlement data logs around the base, as well as low grade engineering materials and a data point that gives data materials. There is an abandoned SRV around 10km away next to a barnacle. It has a few pieces of cargo and can cause Thargoid encounters.
The crashed ship does not have any notable content. The reason for the crash is not obvious – this took place before the first Thargoid War.
Messages
LOG 20TH OF OCTOBER 3111
It’s Friday the 20th of October, 3111. This is Professor Penelope Carver. I’m making this log for our own records, and keeping it separate from the official logs we’re sending to our employer.
Why? Well, I’m still concerned about working for Azimuth Biochemicals, to be honest. Obviously we take on all sorts of contracts for a living, and this isn’t our first top secret commercial project. But the rumours about this corporation… anyway, my people can handle anything, I’m sure. Azimuth are certainly paying well for our skills, I’ll give them that. And those debts of mine aren’t going away by themselves.
The Coalsack Nebula has turned out to have a lot more scientific value than I expected. We established a site on this planet on the 13th, after we detected some very odd signals, and the whole team is excited about what we found. That’s another reason I’m logging this – if this turns out to be a big discovery, I want our names attached!
The things growing here are like nothing on record. Definitely organic life, but with crystalline or metallic elements, and chemistry that even O’Keefe can’t make sense of. They’re giving off signal bursts on all sorts of frequencies.
Are they living organisms or machines of some kind? Murphy and Sheng both think they’re alien in origin – as in, constructed by non—humans – but Kumalo found that so hilarious we had to turn off his comms.
Our transport ship is long gone by now, but Taylor’s using the beacon to transmit our findings back to the company in the Chukchan system. She said the built-in encryption software is top of the line, which makes me think the stories about Azimuth and its rivals might be true. They obviously want to keep whatever we find all to them –
Something’s going on. I can hear everyone shouting. Did the scouting party find something?
LOG 27ND OF OCTOBER 3111
This is Professor Carver, 27th of October, 3111. Hopefully this log will record – half of our equipment isn’t working properly right now.
Everything’s changed since we found the crashed ship. For one thing, it’s… it’s alien. Definitely non-human technology. I should sound more excited, I know. Every scientist’s dream, isn’t it? But something about this just feels wrong. I know the others feel the same. Even Kumalo isn’t smiling any more.
We’re still mostly in the dark about the object we retrieved from inside the wreckage. Is it the pilot, or the cargo, or maybe some organic component of the ship itself? It seems totally inert, but… we’ve had electromagnetic interference all over the base since we brought it back, and that can’t be a coincidence.
Storing it has caused problems. Sheng lashed something together using the strongest bulkheads he could find, but apparently it’s still breaking that down. The equipment failures meant O’Keefe couldn’t fully analyse its chemical structure, making him so angry that he actually attacked Kumalo for telling him to calm down. I’ve never had to break up my people like that – we’ve worked together for years.
It’s Murphy I’m most worried about, though. He can’t tear himself away from those growths on the surface. ‘The stones’, he calls them, like the Neolithic standing stones on Earth. We keep finding him out there in his suit, standing under the jet—black sky, staring at them. He won’t tell us why.
We’ve been sending back reports, but Taylor just relayed a message that made my blood run cold. Azimuth has ordered us to cease all transmissions and await the arrival of their megaship, the Adamastor, which will transport us and our samples to a ‘secure facility’.
And now I’m wondering why I got involved with a corporation that’s rumoured to have its own private army. Have I put everyone at risk by taking this job?
LOG 30TH OF OCTOBER 3111
I’m going to try making this log again, while the data drives are still functioning. They could go again at any time. Nothing works properly anymore. Especially not us. It’s the 30th of October. The object in our storage cage has become… noisy. l have a suspicion it’s even noisier on infrasonic frequencies, which would explain why everyone is on a knife’s edge and unable to sleep. I would ask Mahmood to do some studies, but he’s in the lnfirmary after his run-in with Kumalo. God, I’ve never seen my team like this. What’s wrong with them?
But that’s not the important thing. I don’t think the ship that crashed was alone.
Yesterday, O’Keefe came bursting in through the airlock like a pack of wolves was after him. He said there were lights shining down on the growths and on Murphy, who was just standing there. He couldn’t make out where they came from, only that the nebula above us seemed to be moving. He said… he said the night was opening up like a giant mouth.
I don’t know where Taylor’s got to, so I tried the comms beacon myself, and found a message saying the Adamastor has arrived in the system. That should be a relief, but actually it twists my gut.
What are Azimuth going to do with the thing we found? What are they going to do with us?
The final log seems like it should be given from the Wrecked SRV, but is actually found in the settlement
LOG…
It’s working… suit link is working. Can anyone hear me? Is anyone still back at base? This is… I don’t remember. I’m not – Dominic! Dominic Murphy. That’s who I am, yes. This is Dr Murphy reporting. I’m a geologist, part of Professor Carver’s planetary survey team. But… I think they’re all dead.
I was out here with the stones when the Sidewinders arrived. I watched them come down like birds with broken wings, lights flickering on and off, just like the base has been for days. Then there were soldiers everywhere, with rifles and armoured suits. I think they loaded the cage onto one of the ships, which took off again, just about.
And then a few of the soldiers… I saw the flashes inside the buildings. Gunfire. Something exploded – the main comms relay, I think. But then came more lights, strange lights that rippled like snakes, and… and that blackness again, opening up in the sky.
They tried to get away on foot, running out to where I was hiding, and I thought they’d see me, but then they were all flying up, little toy soldiers thrown in the air, pulled up into the lights…
Now it’s just us. Me and the stones. They’re all I can hear, much louder now, but not hurting like people’s voices used to. Loud but far away. So far…
The site was originally found by JManis following clues that led to the Coalsack.
+Chukchan,142.65625 , 56.46875 , 40.34375, 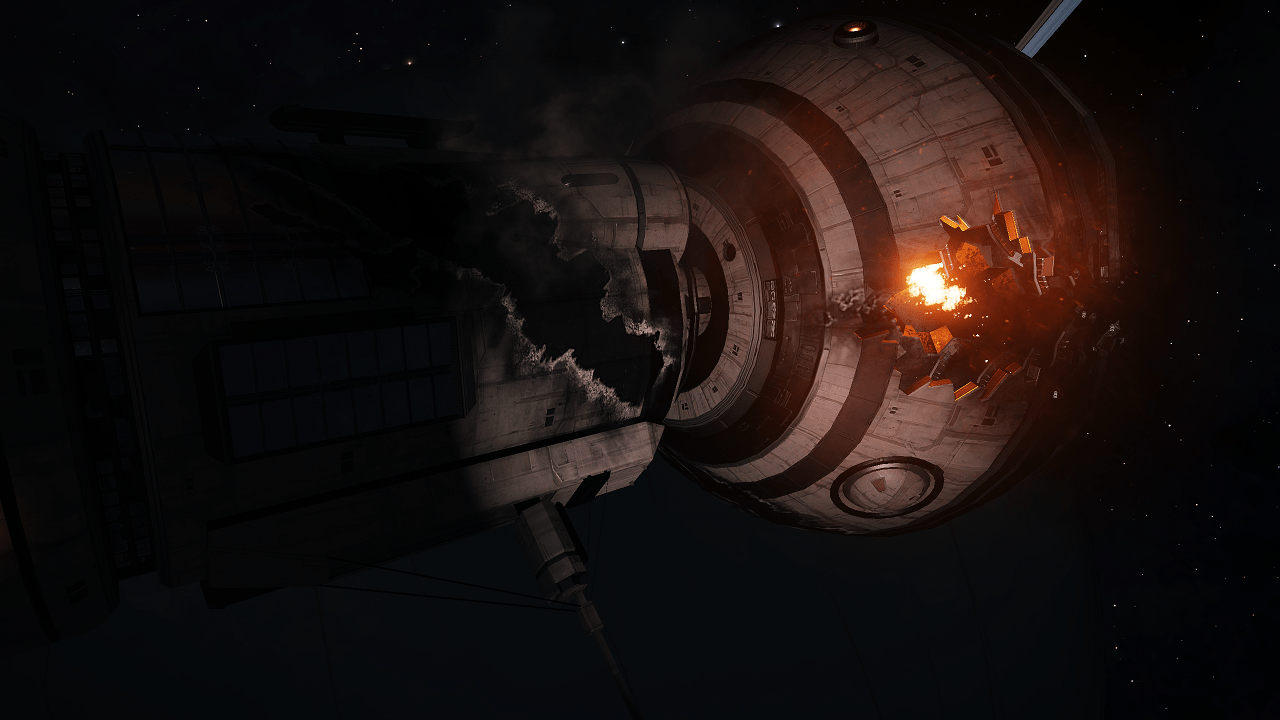
The Adamastor, a Lowell class science megaship, can be found orbiting Chukchan 5 b. It is on fire and there are cargo and engineering materials nearby. Scanning the ship with the Datalink scanner reveals the usual array of subtargets, including a data point that gives more information about the ship. There is also a local news article about the ship, which can be found in the newsfeeds of local stations (this will probably expire at some point).
Arrival of the Adamastor
The arrival of the Adamastor was reported in October 3306 in a Galnet article:
‘Ghost Ship’ Arrives in Chukchan System
29 OCT 3306
Pilots Federation ALERT
An abandoned megaship that set out two centuries ago has returned on automatic pilot to the Chukchan system.
Local security forces were scrambled to intercept an unidentified vessel that was detected on a collision course with Chukchan 5 B.
The megaship displayed no signs of life, so tugs were deployed to wrench it into a safe orbit. This caused onboard power systems to overload with explosive results, damaging cargo holds and spilling debris.
The name of the ship was confirmed as the Adamastor, but no further information is available in the public domain.
Specialists from the Alliance Salvage Guild boarded the derelict to conduct an investigation. Their preliminary report stated:
“The Adamastor has been travelling at sublight speed through interstellar space for nearly two hundred years. There are no remains of the crew. All escape pods and secondary craft have been launched, and several airlocks were manually opened from within.”
“A complete absence of identification suggests that this ship was intended for clandestine operations. All data drives have been deliberately erased.”
“There is evidence of a reinforced cargo hold, fitted with what looks like the remains of environmental equipment. Recent fire damage makes it impossible to be more specific.”
“The ship’s original destination is unknown, having returned to its launch point on autopilot. Calculating its trajectory implies that it passed near the HIP 61595, HIP 64011 and HIP 65201 systems.”
The Adamastor will remain in orbit for further examination.
Further information was found in the local news article:
SECRETS OF THE ADAMASTOR
29 OCT 3306
A private investigator has uncovered some background to the derelict megaship that recently arrived in this system.
Little is known about the Adamastor, which returned on autopilot after two hundred years with no sign of its crew. But an experienced detective has come out of retirement to tackle this mystery.
Local resident Benjamin Chester, who was a founding member of the renowned Wallglass Investigations Agency, told journalists:
“I convinced the Alliance Salvage Guild to pass on some serial numbers that they discovered inside the Adamastor. Then I started scouring databases, interviewing sources and, well, doing my old job.” “The megaship was owned by a corporation called Azimuth Biochemicals. This was taken over by a similar company called Pharmasapien, but both were liquidated long ago. No mission records exist, but service crew reports mention that the Adamastor’s passengers included scientists, engineers and some kind of military.”
“Eventually I unearthed a flight plan, logged in October 3111. It plotted a course toward Barnard’s Loop with fuel for approximately 370 light years, and was scheduled to pass by the HIP 39748 and HIP 33386 systems.”
“I’m way too old to head out there myself, but I hope someone can use this info, and help satisfy my curiosity about what happened all those years ago.”
Scanning the datapoint on the Adamastor itself reveals yet more information:
LOG
Log: 26 10 3111:
Departing under private licence.
Log: 28 …:
…
Log: 29 10 3111:
… Entering system with a single K class star and 11 bodies. Heading for third body. Arrived at listening post. Updating route to rendezvous with planetary survey team.
Log: 30 10 3111:
Entering system with a single M class star. Heading… metal body… Outpost latitude 11.86… Retrieve… cargo… Emergency de… initiated…
Log: 31 10 3111:
Currently 1.. Ly
…
Entering system with single A class star…
Security system offl…
Ship lau… … …
Flight of the Adamastor
From the local news article it was determined that the Adamastor had set out from Chukchan in 3111 bound for the Barnard’s Loop area on a mission for Azimuth Biochemicals. It was heading along a path through HIP 39748 and HIP 33386 and was expected to travel 370 Ly. The Adamastor log indicated that the first stop was at a system with a single K class star with 11 other bodies. A Listening Post was found in Synuefe XE-Y C17-7 that matches this (at this time the message from the Listening Post has not been decoded).
It is believed that the message at the Listening Post caused the Adamastor to be redirected to the Coalsack Nebula to Site 23B in Musca Dark Region PJ-P B6-1 (which is a system that matches the description in the Adamastor message on 30 10 3111). Site 23B had discovered a crashed Thargoid Ship with an alien artefact – believed to be either a Thargoid Probe or Sensor. This was placed aboard the Adamastor for delivery back to Azimuth Biochemicals at an unknown location. As this was an early contact with Thargoid Technology they did not have sufficient shielding to prevent the alien technology interfering with the ship. As the Adamastor was travelling through HIP 69200 Professor Carver from the Site 23 expedition left the Adamastor in a Sidewinder in an attempt to escape, as she believed the Adamastor was doomed. Her crash can be found in this system. HIP 69200 is the system referenced in the 31 10 3111 log on the Adamastor, it is 178 Ly from Chukchan, which matches the ‘1.. Ly’ part of the message, and is at the end of a path going through Chukchan and the systems named in Galnet.
It is assumed that soon after this event the Adamastor was critically damaged and took an autopilot course back to Chukchan rather than the intended destination (its path from Site 23B does not head to Chukchan). It passed through the three systems named in the Galnet article (HIP 61595, HIP 64011 and HIP 65201) and arrived back in Chukchan around 200 years after setting out – presumably due to being unable to use hyperspace travel.
+AE Aurigae, -246.71875 , -54.6875 , -1857.40625, related to Barnard's Loop
+Mu Columbae, 1001.6875 , -598.625 ,-640.78125, related to Barnard's Loop
+53 Arietis, -196.84375 , -461.09375 , -664.0625 , related to Barnard's Loop
+HIP 69200,170.96875 , 113.15625 , 207.15625, 
Dr Carver escapes in sidewinder and crashes
This is Professor Carver.
I’ve just got time to record this before the others join me. In case we don’t make it. Things went to hell on the Adamastor much faster than before. When it left orbit without picking up the rest of my team, we were furious. Weirdly, so were some of the soldiers — mercenaries, just like the rumours said. They demanded to know why half their squad had been left behind, but the bridge crew refused to explain anything.
Then the power fluctuations started. Sheng, O’Keefe and I knew what was coming. The cage they’ve constructed in the cargo hold isn’t going to be strong enough. Even the Adamastor’s hyperdrive keeps failing. They’re making emergency repairs to it now while we’re passing through some unexplored system, with half the ship in darkness.
This sounds crazy but even though the nebula is three hundred light years behind us, it feels like it’s reaching out, trying to drag us back… swallow us whole.
When all the security systems crashed, I took my chance and broke into one of the Sidewinders. We’re going to jump ship. I need to get my people out. The few who are left. I’m the reason they’re here, my stupid, selfish greed.
I can hear screaming. Shooting. It’s happening again! Where’s Sheng and O’Keefe? I think the hyperdrive is coming back online – I need to launch! Damn it, where are they?
I’m sorry. I have to get out. Oh God… I’m so sorry…
+Extention1,-2048.46875 ,3826.59375 ,7786.8125, Point extending the line between HIP 39748 and IP 33386
+Extention2,2395.28125, -3823.40625, -7913.1875, Point extending the line between HIP 39748 and IP 33386
+HIP 61595,146.71875 , 67.9375 , 86.8125
+HIP 64011,148.59375 , 85.5625 , 111.625
+HIP 65201,157.34375 , 86.375 , 131.40625
+Route Intersection, 100 , 144 , 184,Extending the route from the Musca Dark Region PJ-P B6-1 through HIP 69200 and extending the line from the Barnard's Loop route backwards approximates to the position of Col 285 Sector TK-D c13-11. There are some theories that the Adamastor was on its way to this region of space when it was crippled and returned on autopilot at sub light speed to Chukchan. However it has also been pointed out that Chukchan is the begining of the journey and the corporate HQ so there may be some doubt as to the exact position or even if there is anything to be found at all.
+Col 285 Sector LK-I b25-3,83.625,139.8125,180.375
+Col 285 Sector JP-I b25-0,86,155.25,184.65625
+Col 285 Sector TK-D c13-16,87.75,150.21875,183.6875
+Col 285 Sector TK-D c13-11,90.46875,154.375,179
+Col 285 Sector LK-I b25-5,91.4375,151.96875,184.875
+HIP 70991,93.78125,154.75,186.5
+HIP 70768,94.625,131.96875,169.8125
+Col 285 Sector LK-I b25-4,94.625,151.25,184.75
+Col 285 Sector OV-G b26-3,95.65625,156,198.9375
+Col 285 Sector QQ-G b26-1,95.8125,137.8125,198.75
+Col 285 Sector IE-K b24-1,98.875,148.96875,166.5625
+Col 285 Sector WF-D c13-4,99.71875,133.84375,178.15625
+Col 285 Sector WF-D c13-9,99.84375,131.03125,197.71875
+Col 285 Sector QQ-G b26-0,99.84375,135.34375,200.75
+Col 285 Sector UK-D c13-10,100.09375,146.96875,202
+Col 285 Sector QQ-G b26-2,100.59375,141.78125,200.34375
+Col 285 Sector OF-I b25-2,101.65625,134.15625,182.96875
+Col 285 Sector YP-O d6-85,101.78125,127.1875,178.46875
+Col 285 Sector WF-D c13-5,101.8125,132.71875,176.8125
+Col 285 Sector MK-I b25-5,101.96875,144.21875,184.28125
+Col 285 Sector QQ-G b26-3,102.84375,136.1875,196.59375
+Col 285 Sector WF-D c13-3,103,131.09375,176.59375
+Col 285 Sector UK-D c13-9,103.375,142.0625,199.03125
+Col 285 Sector KZ-J b24-5,103.78125,133.3125,173.375
+MW Virginis,104.65625,162.125,179.03125
+HIP 70776,106.96875,149.46875,192.3125
+Col 285 Sector MK-I b25-0,107.46875,150.96875,187.125
+Col 285 Sector WU-O d6-68,108.59375,156.375,196.59375
+Col 285 Sector MK-I b25-1,109.96875,148.34375,190.625
+Col 285 Sector MK-I b25-4,111.25,135.21875,182.625
+HIP 71019,112.03125,131.625,191.84375
+HIP 69696,113.6875,145.09375,177.0625
+Col 285 Sector MK-I b25-2,114.4375,141.59375,192.09375
+Col 285 Sector MK-I b25-3,114.9375,137.90625,186.25
+Col 285 Sector UK-D c13-11,116.5,147.96875,193.71875
+Col 285 Sector NK-I b25-0,119.875,140.53125,179.25
\ No newline at end of file
diff --git a/data/csvCache/col70.csv b/data/csvCache/col70.csv
new file mode 100644
index 0000000..067500b
--- /dev/null
+++ b/data/csvCache/col70.csv
@@ -0,0 +1,1794 @@
+name,sector,region,pos_x,pos_y,pos_z
+Col 70 Sector AA-D b17-0,Col 70 Sector,Unknown,377.5625,15.28125,-1244.1875
+Col 70 Sector AA-F b28-0,Col 70 Sector,Unknown,376.09375,-279.28125,-999.96875
+Col 70 Sector AA-F b41-0,Col 70 Sector,Unknown,541,-410.125,-718.28125
+Col 70 Sector AA-O b36-0,Col 70 Sector,Unknown,468.3125,-392.90625,-805.78125
+Col 70 Sector AA-V c4-2,Col 70 Sector,Unknown,793.59375,-98.90625,-1419.4375
+Col 70 Sector AB-M b24-0,Col 70 Sector,Unknown,180.65625,-281.21875,-1080.625
+Col 70 Sector AC-T b21-0,Col 70 Sector,Unknown,777.28125,-103.8125,-1139.09375
+Col 70 Sector AD-G c12-0,Col 70 Sector,Unknown,224.03125,-253.5625,-1068
+Col 70 Sector AD-O b37-0,Col 70 Sector,Unknown,259.09375,-203.59375,-788.71875
+Col 70 Sector AD-O c21-3,Col 70 Sector,Unknown,536.78125,-328.03125,-689.34375
+Col 70 Sector AF-E a48-0,Col 70 Sector,Unknown,166.75,-42.5625,-1093.84375
+Col 70 Sector AF-T b22-0,Col 70 Sector,Unknown,593.0625,105.96875,-1123.1875
+Col 70 Sector AH-K b25-0,Col 70 Sector,Unknown,96.09375,-281.625,-1063.1875
+Col 70 Sector AI-G b41-0,Col 70 Sector,Unknown,304.28125,-268.875,-707.3125
+Col 70 Sector AI-H b27-0,Col 70 Sector,Unknown,59.71875,-222.3125,-1019.125
+Col 70 Sector AI-Y b44-0,Col 70 Sector,Unknown,303.3125,-377.34375,-630.28125
+Col 70 Sector AJ-D b43-0,Col 70 Sector,Unknown,260.96875,-221.625,-681.25
+Col 70 Sector AJ-P b23-0,Col 70 Sector,Unknown,577.65625,-125,-1101.59375
+Col 70 Sector AK-P b35-0,Col 70 Sector,Unknown,458.03125,-426.125,-826.28125
+Col 70 Sector AL-D b29-0,Col 70 Sector,Unknown,340.96875,-250.96875,-972.59375
+Col 70 Sector AL-J b40-2,Col 70 Sector,Unknown,671.71875,-138.53125,-728.625
+Col 70 Sector AM-A b31-0,Col 70 Sector,Unknown,296.625,-201.75,-942.875
+Col 70 Sector AM-H b41-0,Col 70 Sector,Unknown,544.78125,-163.8125,-720
+Col 70 Sector AM-K d9-0,Col 70 Sector,Unknown,584.625,-20.96875,-819.6875
+Col 70 Sector AM-L d8-17,Col 70 Sector,Unknown,323.71875,-297.15625,-874.65625
+Col 70 Sector AN-O b37-0,Col 70 Sector,Unknown,347.9375,-146.65625,-787.59375
+Col 70 Sector AO-D b43-1,Col 70 Sector,Unknown,310.53125,-193.875,-670.4375
+Col 70 Sector AP-R d4-3,Col 70 Sector,Unknown,761.1875,-696.59375,-1202.375
+Col 70 Sector AP-Z d1,Col 70 Sector,Unknown,741.09375,-328,-1538.96875
+Col 70 Sector AR-H b41-0,Col 70 Sector,Unknown,578.5,-143.4375,-721.96875
+Col 70 Sector AS-G c12-5,Col 70 Sector,Unknown,475.75,-131.4375,-1093.78125
+Col 70 Sector AS-R b22-1,Col 70 Sector,Unknown,790.125,-60.46875,-1116.34375
+Col 70 Sector AT-O b36-0,Col 70 Sector,Unknown,101.6875,-316.46875,-809.1875
+Col 70 Sector AU-B c15-1,Col 70 Sector,Unknown,149.125,-45.3125,-961.28125
+Col 70 Sector AU-Q c5-7,Col 70 Sector,Unknown,619.28125,-593.84375,-1347.53125
+Col 70 Sector AU-S c19-5,Col 70 Sector,Unknown,322.5,-83.8125,-757.21875
+Col 70 Sector AV-N b36-0,Col 70 Sector,Unknown,415.9375,-420,-822.75
+Col 70 Sector AV-P b9-0,Col 70 Sector,Unknown,737.78125,-121.65625,-1399.6875
+Col 70 Sector AV-S b22-0,Col 70 Sector,Unknown,497.625,55.71875,-1118.46875
+Col 70 Sector AW-M d7-6,Col 70 Sector,Unknown,274.53125,-477.375,-966.9375
+Col 70 Sector AX-H b27-0,Col 70 Sector,Unknown,179.84375,-163.53125,-1019.84375
+Col 70 Sector AX-J d9-29,Col 70 Sector,Unknown,167.59375,-216.59375,-749.96875
+Col 70 Sector AX-J d9-9,Col 70 Sector,Unknown,132.0625,-228.875,-808.46875
+Col 70 Sector AZ-Q c5-1,Col 70 Sector,Unknown,707.5625,-567,-1378.9375
+Col 70 Sector BA-W c3-12,Col 70 Sector,Unknown,666.09375,-237.3125,-1430.09375
+Col 70 Sector BA-W c3-8,Col 70 Sector,Unknown,670.46875,-260.96875,-1427.59375
+Col 70 Sector BA-Y d1-0,Col 70 Sector,Unknown,675.0625,-211.21875,-1389.9375
+Col 70 Sector BA-Y d1-6,Col 70 Sector,Unknown,669.125,-214.03125,-1464.9375
+Col 70 Sector BB-J a59-1,Col 70 Sector,Unknown,215.71875,-45,-972.15625
+Col 70 Sector BB-K c10-3,Col 70 Sector,Unknown,744.59375,-146.0625,-1171.40625
+Col 70 Sector BB-M b24-0,Col 70 Sector,Unknown,197.09375,-282.09375,-1080.40625
+Col 70 Sector BB-V d3-30,Col 70 Sector,Unknown,562.78125,50.875,-1234.75
+Col 70 Sector BC-D a35-0,Col 70 Sector,Unknown,237.40625,-32.0625,-1234.5625
+Col 70 Sector BC-I b27-0,Col 70 Sector,Unknown,250.3125,-142.71875,-1005.28125
+Col 70 Sector BC-K b25-0,Col 70 Sector,Unknown,82.4375,-285.125,-1055.5625
+Col 70 Sector BD-F b42-0,Col 70 Sector,Unknown,358.9375,-222.59375,-701.96875
+Col 70 Sector BD-O b37-0,Col 70 Sector,Unknown,277.15625,-201.6875,-804.71875
+Col 70 Sector BD-X b45-0,Col 70 Sector,Unknown,374.28125,-314.34375,-624.375
+Col 70 Sector BE-D b43-0,Col 70 Sector,Unknown,235.71875,-241.40625,-680.5
+Col 70 Sector BE-U b6-0,Col 70 Sector,Unknown,799.96875,-220.09375,-1459.15625
+Col 70 Sector BF-G b27-0,Col 70 Sector,Unknown,357.5625,-344.6875,-1023.5
+Col 70 Sector BF-W c3-1,Col 70 Sector,Unknown,772.3125,-221.40625,-1463.5
+Col 70 Sector BF-X b31-0,Col 70 Sector,Unknown,436.21875,-363,-919.625
+Col 70 Sector BG-F b40-0,Col 70 Sector,Unknown,320.46875,-581.28125,-743.59375
+Col 70 Sector BG-M b24-0,Col 70 Sector,Unknown,239.75,-260.40625,-1082.09375
+Col 70 Sector BG-V b32-0,Col 70 Sector,Unknown,319.90625,-381.3125,-899.625
+Col 70 Sector BH-Q a55-1,Col 70 Sector,Unknown,137.53125,-31.4375,-1011.28125
+Col 70 Sector BI-F c13-2,Col 70 Sector,Unknown,529.96875,-44.90625,-1032.875
+Col 70 Sector BK-O c7-0,Col 70 Sector,Unknown,815,-381.9375,-1299.78125
+Col 70 Sector BK-P b35-0,Col 70 Sector,Unknown,476.1875,-439.875,-844.46875
+Col 70 Sector BK-Z d0,Col 70 Sector,Unknown,686.8125,-392.5625,-1532.1875
+Col 70 Sector BL-L b38-0,Col 70 Sector,Unknown,516.28125,-286.90625,-774.8125
+Col 70 Sector BL-M b37-0,Col 70 Sector,Unknown,453.375,-377.53125,-801.59375
+Col 70 Sector BM-K d9-13,Col 70 Sector,Unknown,658.5625,-3.0625,-797.5
+Col 70 Sector BM-S b21-0,Col 70 Sector,Unknown,167.34375,-153.625,-1125.53125
+Col 70 Sector BN-I b26-0,Col 70 Sector,Unknown,39.09375,-281.8125,-1038.8125
+Col 70 Sector BQ-M b37-0,Col 70 Sector,Unknown,476.96875,-363.46875,-800.125
+Col 70 Sector BQ-W d2-20,Col 70 Sector,Unknown,674.90625,-65.28125,-1315.53125
+Col 70 Sector BR-Y a64-1,Col 70 Sector,Unknown,259.71875,-32.46875,-911
+Col 70 Sector BS-I b39-0,Col 70 Sector,Unknown,240.9375,-386.9375,-761.53125
+Col 70 Sector BT-D c14-8,Col 70 Sector,Unknown,425.78125,4.65625,-987.6875
+Col 70 Sector BT-E c13-3,Col 70 Sector,Unknown,271.65625,-146.1875,-1061.28125
+Col 70 Sector BT-O b36-0,Col 70 Sector,Unknown,125.1875,-310.96875,-819.875
+Col 70 Sector BU-C b15-0,Col 70 Sector,Unknown,796.0625,-381.875,-1279.34375
+Col 70 Sector BU-H b26-0,Col 70 Sector,Unknown,397.15625,-363.78125,-1043.03125
+Col 70 Sector BU-H c10-5,Col 70 Sector,Unknown,816.3125,-626.78125,-1175.46875
+Col 70 Sector BU-H c10-9,Col 70 Sector,Unknown,819.03125,-626,-1148.4375
+Col 70 Sector BU-Y c1-0,Col 70 Sector,Unknown,659.3125,-403.96875,-1538.65625
+Col 70 Sector BU-Z c0,Col 70 Sector,Unknown,518.03125,-554.25,-1556.6875
+Col 70 Sector BV-N b36-0,Col 70 Sector,Unknown,436.59375,-410.3125,-821.21875
+Col 70 Sector BW-A b18-0,Col 70 Sector,Unknown,238.15625,-24.75,-1220.6875
+Col 70 Sector BW-M d7-3,Col 70 Sector,Unknown,341.40625,-444.59375,-965.09375
+Col 70 Sector BW-M d7-6,Col 70 Sector,Unknown,414.125,-435.34375,-948.09375
+Col 70 Sector BX-I b26-0,Col 70 Sector,Unknown,117.59375,-243.53125,-1029.53125
+Col 70 Sector BX-Q b35-0,Col 70 Sector,Unknown,278.375,-270.03125,-840.59375
+Col 70 Sector BX-R b21-0,Col 70 Sector,Unknown,52.3125,-219.75,-1141.46875
+Col 70 Sector BY-F c12-1,Col 70 Sector,Unknown,214,-290.71875,-1099.8125
+Col 70 Sector CA-Q d5-9,Col 70 Sector,Unknown,763.84375,-618.5,-1093.375
+Col 70 Sector CB-A b32-1,Col 70 Sector,Unknown,535.78125,-51.03125,-913.8125
+Col 70 Sector CE-E c13-0,Col 70 Sector,Unknown,61.09375,-274.34375,-1037.4375
+Col 70 Sector CE-E c13-1,Col 70 Sector,Unknown,66.125,-282.59375,-1050.34375
+Col 70 Sector CE-F b16-0,Col 70 Sector,Unknown,575.46875,56.65625,-1263.15625
+Col 70 Sector CE-N b37-0,Col 70 Sector,Unknown,95.9375,-302.96875,-799.6875
+Col 70 Sector CF-P c6-1,Col 70 Sector,Unknown,620,-575.09375,-1342.15625
+Col 70 Sector CF-P c6-9,Col 70 Sector,Unknown,633.375,-561.625,-1333.6875
+Col 70 Sector CH-L d8-2,Col 70 Sector,Unknown,276.28125,-363.3125,-847.5
+Col 70 Sector CH-W b31-0,Col 70 Sector,Unknown,775.65625,-460.21875,-921.65625
+Col 70 Sector CI-G b28-0,Col 70 Sector,Unknown,176.96875,-139.8125,-1004.6875
+Col 70 Sector CI-P b36-0,Col 70 Sector,Unknown,260.25,-263.875,-823.25
+Col 70 Sector CJ-E b42-0,Col 70 Sector,Unknown,216.25,-295.78125,-702.78125
+Col 70 Sector CK-H c10-0,Col 70 Sector,Unknown,699.3125,-725.875,-1153.15625
+Col 70 Sector CM-H b41-1,Col 70 Sector,Unknown,576.125,-155.71875,-708.90625
+Col 70 Sector CM-H b41-2,Col 70 Sector,Unknown,580.5625,-154.5,-715.875
+Col 70 Sector CP-B b3-0,Col 70 Sector,Unknown,695.25,-181.21875,-1526.875
+Col 70 Sector CP-G b27-0,Col 70 Sector,Unknown,458.96875,-299.3125,-1024.96875
+Col 70 Sector CP-Q c5-2,Col 70 Sector,Unknown,625.96875,-626.21875,-1359.6875
+Col 70 Sector CP-Q c5-8,Col 70 Sector,Unknown,635.125,-625.6875,-1374.15625
+Col 70 Sector CQ-N b36-0,Col 70 Sector,Unknown,420.3125,-439.78125,-824.75
+Col 70 Sector CR-I c11-4,Col 70 Sector,Unknown,785.125,-83.375,-1135.6875
+Col 70 Sector CR-P b34-0,Col 70 Sector,Unknown,780.625,-603.78125,-862.78125
+Col 70 Sector CS-J d9-13,Col 70 Sector,Unknown,111.0625,-308.8125,-816
+Col 70 Sector CT-O c20-0,Col 70 Sector,Unknown,299.78125,-582.0625,-741.84375
+Col 70 Sector CT-W b45-0,Col 70 Sector,Unknown,300,-364.28125,-624.625
+Col 70 Sector CU-H b26-0,Col 70 Sector,Unknown,424.625,-363,-1044.34375
+Col 70 Sector CU-K c23-5,Col 70 Sector,Unknown,381.75,-297.21875,-616.84375
+Col 70 Sector CU-Q c5-3,Col 70 Sector,Unknown,707.15625,-595.46875,-1384.84375
+Col 70 Sector CU-Q c5-7,Col 70 Sector,Unknown,700.78125,-609.6875,-1374.625
+Col 70 Sector CU-Y c1-0,Col 70 Sector,Unknown,699.25,-422.40625,-1541.6875
+Col 70 Sector CV-N b36-0,Col 70 Sector,Unknown,459.4375,-412.5625,-823.5625
+Col 70 Sector CW-F b27-0,Col 70 Sector,Unknown,818.71875,-383.21875,-1010.125
+Col 70 Sector CX-I b26-0,Col 70 Sector,Unknown,139.75,-239.65625,-1040.71875
+Col 70 Sector CX-Q b22-0,Col 70 Sector,Unknown,152.6875,-130.25,-1115.0625
+Col 70 Sector CY-E b42-0,Col 70 Sector,Unknown,340.65625,-240.90625,-699.9375
+Col 70 Sector CY-W b45-0,Col 70 Sector,Unknown,350.34375,-331.75,-624.4375
+Col 70 Sector CZ-C b43-0,Col 70 Sector,Unknown,216.78125,-261.53125,-683.75
+Col 70 Sector DA-Q d5-13,Col 70 Sector,Unknown,817.6875,-622.625,-1073.34375
+Col 70 Sector DA-Q d5-8,Col 70 Sector,Unknown,817.28125,-626.9375,-1121.625
+Col 70 Sector DB-T c5-3,Col 70 Sector,Unknown,692.15625,-137.28125,-1374.90625
+Col 70 Sector DC-R c19-3,Col 70 Sector,Unknown,735.875,-456.78125,-754.75
+Col 70 Sector DC-S b21-0,Col 70 Sector,Unknown,134.125,-187.34375,-1132.78125
+Col 70 Sector DC-T d4-3,Col 70 Sector,Unknown,193.6875,-89,-1224.5
+Col 70 Sector DD-G b41-0,Col 70 Sector,Unknown,329.75,-296.5,-706
+Col 70 Sector DD-H b27-0,Col 70 Sector,Unknown,76.875,-241.0625,-1019.0625
+Col 70 Sector DF-G b27-0,Col 70 Sector,Unknown,397.8125,-341.125,-1023.90625
+Col 70 Sector DF-L b39-0,Col 70 Sector,Unknown,807.625,-129.3125,-760.5
+Col 70 Sector DF-O c7-3,Col 70 Sector,Unknown,818.625,-419.6875,-1273.25
+Col 70 Sector DF-O c7-4,Col 70 Sector,Unknown,819.65625,-419.9375,-1273.03125
+Col 70 Sector DF-O c7-8,Col 70 Sector,Unknown,816,-413.9375,-1293.5625
+Col 70 Sector DF-Y c1-0,Col 70 Sector,Unknown,498,-540.625,-1543.78125
+Col 70 Sector DF-Z d1,Col 70 Sector,Unknown,702.34375,-492.71875,-1488.5
+Col 70 Sector DF-Z d2,Col 70 Sector,Unknown,684.0625,-458.03125,-1498.6875
+Col 70 Sector DF-Z d3,Col 70 Sector,Unknown,697.21875,-489.75,-1516.03125
+Col 70 Sector DF-Z d4,Col 70 Sector,Unknown,705.3125,-484.90625,-1468.8125
+Col 70 Sector DG-C b43-0,Col 70 Sector,Unknown,607.84375,-326.28125,-683.84375
+Col 70 Sector DH-H b41-1,Col 70 Sector,Unknown,555.5625,-182.90625,-723.4375
+Col 70 Sector DH-T d4-24,Col 70 Sector,Unknown,381.90625,20.09375,-1175.09375
+Col 70 Sector DH-T d4-6,Col 70 Sector,Unknown,386.40625,21.375,-1207.84375
+Col 70 Sector DI-P b23-0,Col 70 Sector,Unknown,130.4375,-116.84375,-1096.84375
+Col 70 Sector DJ-S c4-10,Col 70 Sector,Unknown,820.65625,-626.4375,-1405.90625
+Col 70 Sector DJ-S c4-7,Col 70 Sector,Unknown,822.375,-625.71875,-1385.34375
+Col 70 Sector DK-G b27-0,Col 70 Sector,Unknown,450.5625,-312.1875,-1009.625
+Col 70 Sector DK-Q b34-0,Col 70 Sector,Unknown,435.1875,-524.84375,-855.09375
+Col 70 Sector DK-X c2-0,Col 70 Sector,Unknown,748.25,-325.125,-1481.875
+Col 70 Sector DL-C a49-0,Col 70 Sector,Unknown,159.09375,-38.9375,-1075.65625
+Col 70 Sector DN-G b41-0,Col 70 Sector,Unknown,409.59375,-257.875,-707.46875
+Col 70 Sector DN-R b22-0,Col 70 Sector,Unknown,798.53125,-80.53125,-1120.75
+Col 70 Sector DO-V b46-1,Col 70 Sector,Unknown,360.46875,-302.625,-603.8125
+Col 70 Sector DP-H b26-0,Col 70 Sector,Unknown,411.34375,-365.40625,-1038.21875
+Col 70 Sector DP-K c23-2,Col 70 Sector,Unknown,342.8125,-344.8125,-613.53125
+Col 70 Sector DP-Q c5-1,Col 70 Sector,Unknown,670.125,-625.9375,-1357.78125
+Col 70 Sector DP-Q c5-3,Col 70 Sector,Unknown,689.34375,-625.5625,-1376.40625
+Col 70 Sector DP-Q c5-4,Col 70 Sector,Unknown,692.875,-625.59375,-1377.84375
+Col 70 Sector DP-Q c5-6,Col 70 Sector,Unknown,683.75,-625.21875,-1381
+Col 70 Sector DP-Q c5-8,Col 70 Sector,Unknown,656.125,-625.1875,-1373.09375
+Col 70 Sector DP-Y c1-0,Col 70 Sector,Unknown,674.875,-447.65625,-1527.3125
+Col 70 Sector DQ-N b36-0,Col 70 Sector,Unknown,435.1875,-444.4375,-820.4375
+Col 70 Sector DR-A b44-1,Col 70 Sector,Unknown,562,-312.90625,-647.1875
+Col 70 Sector DR-H b28-0,Col 70 Sector,Unknown,478.25,-1.78125,-1003.375
+Col 70 Sector DR-H b28-1,Col 70 Sector,Unknown,475.09375,-4.4375,-1002.0625
+Col 70 Sector DS-J d9-14,Col 70 Sector,Unknown,179.875,-326.09375,-755.4375
+Col 70 Sector DS-S b21-0,Col 70 Sector,Unknown,760.34375,-127.5,-1142.8125
+Col 70 Sector DT-J b38-0,Col 70 Sector,Unknown,730.78125,-476.1875,-783.625
+Col 70 Sector DT-Q a41-0,Col 70 Sector,Unknown,237.9375,-32.3125,-1162.0625
+Col 70 Sector DU-Z b29-0,Col 70 Sector,Unknown,436.8125,-449.46875,-954.21875
+Col 70 Sector DW-K b38-0,Col 70 Sector,Unknown,454.375,-361.1875,-769.84375
+Col 70 Sector DW-L b24-0,Col 70 Sector,Unknown,199.1875,-300.5625,-1080.40625
+Col 70 Sector DX-I b26-0,Col 70 Sector,Unknown,157.9375,-236.65625,-1027.34375
+Col 70 Sector DX-R b21-0,Col 70 Sector,Unknown,80.59375,-223.09375,-1144.4375
+Col 70 Sector DY-E b42-0,Col 70 Sector,Unknown,356.9375,-234.3125,-704.625
+Col 70 Sector DY-N b37-0,Col 70 Sector,Unknown,284.5,-208.28125,-799.59375
+Col 70 Sector DY-W b45-0,Col 70 Sector,Unknown,356.90625,-341.25,-625
+Col 70 Sector DZ-F a47-0,Col 70 Sector,Unknown,236.875,-44.90625,-1102.1875
+Col 70 Sector EA-M b25-1,Col 70 Sector,Unknown,538.84375,-89.0625,-1049.125
+Col 70 Sector EA-P c6-4,Col 70 Sector,Unknown,618.8125,-614.03125,-1335.03125
+Col 70 Sector EA-P c6-6,Col 70 Sector,Unknown,619.375,-612.75,-1343.84375
+Col 70 Sector EA-P c6-7,Col 70 Sector,Unknown,648.75,-618.3125,-1338.21875
+Col 70 Sector EA-S c19-4,Col 70 Sector,Unknown,144.6875,-243.40625,-772.625
+Col 70 Sector EB-C b30-0,Col 70 Sector,Unknown,425.71875,-224.6875,-948.59375
+Col 70 Sector EC-H c12-2,Col 70 Sector,Unknown,791.90625,-43.21875,-1103.53125
+Col 70 Sector EC-H c12-3,Col 70 Sector,Unknown,785,-48.71875,-1102.4375
+Col 70 Sector ED-H b27-0,Col 70 Sector,Unknown,99.1875,-242.03125,-1020.21875
+Col 70 Sector EG-B b18-0,Col 70 Sector,Unknown,379.90625,17.1875,-1224.0625
+Col 70 Sector EH-K d9-1,Col 70 Sector,Unknown,746,-103.96875,-816.40625
+Col 70 Sector EH-K d9-12,Col 70 Sector,Unknown,781.65625,-76.40625,-775.75
+Col 70 Sector EH-K d9-26,Col 70 Sector,Unknown,783.875,-91.875,-770.46875
+Col 70 Sector EH-K d9-27,Col 70 Sector,Unknown,779.75,-97.90625,-802.34375
+Col 70 Sector EH-K d9-28,Col 70 Sector,Unknown,771.84375,-103.65625,-790.53125
+Col 70 Sector EH-K d9-34,Col 70 Sector,Unknown,793.5,-96.625,-787.0625
+Col 70 Sector EH-K d9-37,Col 70 Sector,Unknown,773.21875,-84.15625,-761.125
+Col 70 Sector EH-K d9-44,Col 70 Sector,Unknown,754.25,-98.125,-819.5
+Col 70 Sector EH-K d9-48,Col 70 Sector,Unknown,772,-100.1875,-764.1875
+Col 70 Sector EH-K d9-56,Col 70 Sector,Unknown,746.5625,-103.5625,-818.28125
+Col 70 Sector EH-L d8-10,Col 70 Sector,Unknown,438.34375,-406.5625,-896.625
+Col 70 Sector EH-L d8-12,Col 70 Sector,Unknown,435.5625,-418.4375,-835.5
+Col 70 Sector EH-L d8-13,Col 70 Sector,Unknown,423.84375,-418.03125,-849.5
+Col 70 Sector EH-L d8-14,Col 70 Sector,Unknown,478.6875,-386.03125,-847.28125
+Col 70 Sector EI-I d10-29,Col 70 Sector,Unknown,312.9375,-161.40625,-688.3125
+Col 70 Sector EI-Y c15-0,Col 70 Sector,Unknown,458.21875,-424.96875,-940.3125
+Col 70 Sector EI-Y c15-1,Col 70 Sector,Unknown,458.8125,-424.5,-942.6875
+Col 70 Sector EK-K c23-1,Col 70 Sector,Unknown,301.75,-380.03125,-620.5625
+Col 70 Sector EK-N b24-0,Col 70 Sector,Unknown,549.5,-128.96875,-1065.9375
+Col 70 Sector EK-T c18-2,Col 70 Sector,Unknown,141.3125,-317.5625,-815.75
+Col 70 Sector EK-X c2-0,Col 70 Sector,Unknown,779.375,-343.28125,-1500.4375
+Col 70 Sector EL-O d6-4,Col 70 Sector,Unknown,771.84375,-563,-1003.3125
+Col 70 Sector EM-T b33-0,Col 70 Sector,Unknown,297.1875,-384.0625,-882.21875
+Col 70 Sector EN-J d9-2,Col 70 Sector,Unknown,131.34375,-365.21875,-759.3125
+Col 70 Sector EO-N b37-0,Col 70 Sector,Unknown,223.96875,-255.4375,-788.21875
+Col 70 Sector EP-N b37-0,Col 70 Sector,Unknown,751.375,-256.34375,-792.28125
+Col 70 Sector EP-S c19-1,Col 70 Sector,Unknown,381.75,-139.40625,-777.8125
+Col 70 Sector EP-Y c1-0,Col 70 Sector,Unknown,703.34375,-445.375,-1525.03125
+Col 70 Sector EQ-K b26-0,Col 70 Sector,Unknown,538.0625,-64.90625,-1043.3125
+Col 70 Sector EQ-N b36-0,Col 70 Sector,Unknown,459.59375,-444,-823.1875
+Col 70 Sector ER-A b44-0,Col 70 Sector,Unknown,586.375,-322.875,-663
+Col 70 Sector ER-B b30-0,Col 70 Sector,Unknown,340.21875,-256.21875,-964.09375
+Col 70 Sector ER-J b39-1,Col 70 Sector,Unknown,511.21875,-287.875,-760.40625
+Col 70 Sector ES-J d9-5,Col 70 Sector,Unknown,311.34375,-333.78125,-763.65625
+Col 70 Sector ES-Y b31-1,Col 70 Sector,Unknown,296.5625,-197.96875,-920.84375
+Col 70 Sector ET-R b34-0,Col 70 Sector,Unknown,739.8125,-365.59375,-851.65625
+Col 70 Sector EV-M c8-1,Col 70 Sector,Unknown,888.96875,-321.03125,-1247.78125
+Col 70 Sector EW-K b38-0,Col 70 Sector,Unknown,455.53125,-361.21875,-781.0625
+Col 70 Sector EX-I b26-0,Col 70 Sector,Unknown,176.15625,-241,-1041.6875
+Col 70 Sector EX-K d8-8,Col 70 Sector,Unknown,153.125,-529,-876.59375
+Col 70 Sector EX-Q c19-2,Col 70 Sector,Unknown,714.65625,-472.34375,-770.3125
+Col 70 Sector EX-R b21-0,Col 70 Sector,Unknown,100.59375,-221.1875,-1142.3125
+Col 70 Sector EY-R b34-0,Col 70 Sector,Unknown,781.40625,-352.25,-850.6875
+Col 70 Sector FA-G b27-0,Col 70 Sector,Unknown,410.875,-358.625,-1024.1875
+Col 70 Sector FA-O c7-1,Col 70 Sector,Unknown,816.125,-437.15625,-1271.78125
+Col 70 Sector FA-S c19-1,Col 70 Sector,Unknown,214.625,-236.1875,-765.09375
+Col 70 Sector FA-X c2-0,Col 70 Sector,Unknown,660.15625,-421.5625,-1501.09375
+Col 70 Sector FA-Y b30-0,Col 70 Sector,Unknown,400.375,-459.34375,-940.34375
+Col 70 Sector FA-Y c1-0,Col 70 Sector,Unknown,496.8125,-554.09375,-1520.40625
+Col 70 Sector FB-M b37-0,Col 70 Sector,Unknown,439.53125,-419.65625,-802.09375
+Col 70 Sector FB-P b9-0,Col 70 Sector,Unknown,693,-187.65625,-1399.78125
+Col 70 Sector FC-I b40-1,Col 70 Sector,Unknown,488.40625,-266.1875,-734.21875
+Col 70 Sector FC-I c11-3,Col 70 Sector,Unknown,667.84375,-206.15625,-1140.71875
+Col 70 Sector FD-I d10-27,Col 70 Sector,Unknown,237.3125,-258.75,-726.96875
+Col 70 Sector FD-I d10-6,Col 70 Sector,Unknown,208.40625,-215,-742.75
+Col 70 Sector FD-Y c15-1,Col 70 Sector,Unknown,424.46875,-462.3125,-940.34375
+Col 70 Sector FE-M c9-4,Col 70 Sector,Unknown,186.625,-75.875,-1208.4375
+Col 70 Sector FE-W c16-0,Col 70 Sector,Unknown,179.625,-499.28125,-902.96875
+Col 70 Sector FE-W c16-1,Col 70 Sector,Unknown,177.25,-486.34375,-904.59375
+Col 70 Sector FG-W d2-18,Col 70 Sector,Unknown,680.625,-237.625,-1376.875
+Col 70 Sector FG-W d2-9,Col 70 Sector,Unknown,665.5625,-231.875,-1350.5625
+Col 70 Sector FI-I d10-9,Col 70 Sector,Unknown,363.1875,-177.21875,-731.15625
+Col 70 Sector FK-B c15-2,Col 70 Sector,Unknown,177.78125,-125.5,-979.8125
+Col 70 Sector FK-S c19-1,Col 70 Sector,Unknown,370.78125,-173.625,-774.125
+Col 70 Sector FK-S c19-2,Col 70 Sector,Unknown,358.59375,-159.375,-780.625
+Col 70 Sector FK-S c19-4,Col 70 Sector,Unknown,362.0625,-158.6875,-768.125
+Col 70 Sector FK-T c18-2,Col 70 Sector,Unknown,182.84375,-331.46875,-789.28125
+Col 70 Sector FM-H b28-0,Col 70 Sector,Unknown,477.65625,-7.125,-1004.46875
+Col 70 Sector FM-H b28-1,Col 70 Sector,Unknown,477.625,-7.09375,-1001.90625
+Col 70 Sector FM-V e2-1,Col 70 Sector,Unknown,99.84375,-230.125,-1118.6875
+Col 70 Sector FN-E b30-1,Col 70 Sector,Unknown,437.9375,42.71875,-962.78125
+Col 70 Sector FN-G c12-3,Col 70 Sector,Unknown,583.625,-164,-1100
+Col 70 Sector FN-Q b22-0,Col 70 Sector,Unknown,130.65625,-183.15625,-1108.09375
+Col 70 Sector FN-U b32-0,Col 70 Sector,Unknown,763.28125,-454.5,-901.53125
+Col 70 Sector FN-V b31-0,Col 70 Sector,Unknown,679.84375,-541.9375,-924.15625
+Col 70 Sector FO-N b37-0,Col 70 Sector,Unknown,247.59375,-254.3125,-803.625
+Col 70 Sector FO-O b36-0,Col 70 Sector,Unknown,157.9375,-325.3125,-815.8125
+Col 70 Sector FP-N b37-0,Col 70 Sector,Unknown,766.8125,-253,-793.78125
+Col 70 Sector FS-B b42-0,Col 70 Sector,Unknown,251.03125,-567.96875,-697.75
+Col 70 Sector FT-F b41-0,Col 70 Sector,Unknown,292.875,-334,-710.1875
+Col 70 Sector FT-W b45-0,Col 70 Sector,Unknown,357.5625,-361.21875,-619.96875
+Col 70 Sector FV-X d1-3,Col 70 Sector,Unknown,867.4375,-332.03125,-1385.6875
+Col 70 Sector FV-X d1-4,Col 70 Sector,Unknown,864.9375,-334.9375,-1385.90625
+Col 70 Sector FW-Q b23-1,Col 70 Sector,Unknown,477.25,45.25,-1099.1875
+Col 70 Sector FX-I b26-0,Col 70 Sector,Unknown,195.28125,-237.4375,-1038
+Col 70 Sector FX-Q c19-2,Col 70 Sector,Unknown,754.8125,-465.28125,-758.78125
+Col 70 Sector FX-R b21-0,Col 70 Sector,Unknown,127.84375,-209.5625,-1143.21875
+Col 70 Sector FY-N c21-1,Col 70 Sector,Unknown,689.28125,-362.1875,-696.8125
+Col 70 Sector FY-N c21-3,Col 70 Sector,Unknown,687.0625,-362.53125,-697.0625
+Col 70 Sector FZ-M b37-0,Col 70 Sector,Unknown,124.125,-314.34375,-797.34375
+Col 70 Sector GB-L c9-6,Col 70 Sector,Unknown,804.625,-337.46875,-1213.78125
+Col 70 Sector GB-M b37-0,Col 70 Sector,Unknown,460.71875,-423.5625,-803.84375
+Col 70 Sector GC-A b31-0,Col 70 Sector,Unknown,336.25,-240.8125,-941.625
+Col 70 Sector GC-I b40-0,Col 70 Sector,Unknown,500.15625,-279.125,-739.21875
+Col 70 Sector GD-I d10-20,Col 70 Sector,Unknown,307.1875,-232.4375,-736.46875
+Col 70 Sector GD-I d10-31,Col 70 Sector,Unknown,332.75,-231.9375,-708
+Col 70 Sector GD-Y c15-0,Col 70 Sector,Unknown,458.34375,-461.5625,-939.4375
+Col 70 Sector GE-D b43-0,Col 70 Sector,Unknown,336,-242.84375,-682.1875
+Col 70 Sector GE-E b42-0,Col 70 Sector,Unknown,256.25,-320.78125,-702.125
+Col 70 Sector GE-I b39-0,Col 70 Sector,Unknown,738.53125,-463.875,-759.5625
+Col 70 Sector GF-Y b30-0,Col 70 Sector,Unknown,459.96875,-442.78125,-941.46875
+Col 70 Sector GG-E c12-6,Col 70 Sector,Unknown,721.34375,-626.28125,-1090.71875
+Col 70 Sector GG-E c12-8,Col 70 Sector,Unknown,697.0625,-625.4375,-1081.34375
+Col 70 Sector GG-W b31-0,Col 70 Sector,Unknown,338.5625,-461.78125,-919.15625
+Col 70 Sector GG-X d1-4,Col 70 Sector,Unknown,452.40625,-576.28125,-1456.125
+Col 70 Sector GG-X e1-3,Col 70 Sector,Unknown,921.71875,-318.78125,-1313.53125
+Col 70 Sector GJ-E b42-0,Col 70 Sector,Unknown,314.6875,-297.4375,-699.8125
+Col 70 Sector GK-Y c1-0,Col 70 Sector,Unknown,697.25,-501.3125,-1540.75
+Col 70 Sector GN-G b41-0,Col 70 Sector,Unknown,458.375,-260.6875,-715.46875
+Col 70 Sector GN-J d9-3,Col 70 Sector,Unknown,265.96875,-370.5,-764.40625
+Col 70 Sector GO-E b42-1,Col 70 Sector,Unknown,350.90625,-266.625,-703.75
+Col 70 Sector GQ-H b13-0,Col 70 Sector,Unknown,817.03125,-242.4375,-1314.78125
+Col 70 Sector GS-K d8-5,Col 70 Sector,Unknown,173.53125,-594.75,-862.21875
+Col 70 Sector GS-Y b18-1,Col 70 Sector,Unknown,179.375,-60.5,-1195.59375
+Col 70 Sector GT-M b38-1,Col 70 Sector,Unknown,380.15625,-156.1875,-773.125
+Col 70 Sector GV-L b25-1,Col 70 Sector,Unknown,537.3125,-111.0625,-1056.0625
+Col 70 Sector GV-X d1-3,Col 70 Sector,Unknown,909.71875,-314.28125,-1395.4375
+Col 70 Sector GW-M d7-9,Col 70 Sector,Unknown,776.4375,-476.25,-977.96875
+Col 70 Sector GX-O b24-1,Col 70 Sector,Unknown,382.03125,21.5,-1070.9375
+Col 70 Sector GY-W b45-0,Col 70 Sector,Unknown,421.34375,-325.03125,-617.59375
+Col 70 Sector GZ-P b35-0,Col 70 Sector,Unknown,697.34375,-379.53125,-839.1875
+Col 70 Sector GZ-V c16-0,Col 70 Sector,Unknown,162.78125,-521.75,-897.9375
+Col 70 Sector HA-H b26-0,Col 70 Sector,Unknown,360.9375,-442.34375,-1040.46875
+Col 70 Sector HD-I d10-14,Col 70 Sector,Unknown,346.09375,-254.875,-694.71875
+Col 70 Sector HD-I d10-4,Col 70 Sector,Unknown,340.4375,-234,-665.3125
+Col 70 Sector HD-I d10-5,Col 70 Sector,Unknown,359.375,-242.8125,-720.625
+Col 70 Sector HD-R d5-7,Col 70 Sector,Unknown,67.8125,-117.875,-1102.65625
+Col 70 Sector HE-Q b35-0,Col 70 Sector,Unknown,756.65625,-362.59375,-844.65625
+Col 70 Sector HG-E c12-8,Col 70 Sector,Unknown,739.78125,-625.15625,-1082.90625
+Col 70 Sector HG-N c7-4,Col 70 Sector,Unknown,576.5625,-587.65625,-1303.6875
+Col 70 Sector HG-X d1-6,Col 70 Sector,Unknown,558.71875,-581.15625,-1401.1875
+Col 70 Sector HJ-Q b22-0,Col 70 Sector,Unknown,651.15625,-196.625,-1117.59375
+Col 70 Sector HL-D b29-0,Col 70 Sector,Unknown,478.40625,-245.8125,-983.875
+Col 70 Sector HL-M c8-4,Col 70 Sector,Unknown,821.4375,-424.21875,-1254.15625
+Col 70 Sector HL-W c2-0,Col 70 Sector,Unknown,497.625,-539.375,-1503.53125
+Col 70 Sector HM-B c14-0,Col 70 Sector,Unknown,757.53125,-487.21875,-993.9375
+Col 70 Sector HN-R d5-18,Col 70 Sector,Unknown,340.28125,48.71875,-1072.09375
+Col 70 Sector HO-O b36-0,Col 70 Sector,Unknown,199.21875,-334.3125,-805.3125
+Col 70 Sector HO-U c18-10,Col 70 Sector,Unknown,754.71875,-99.0625,-823.46875
+Col 70 Sector HP-K c10-1,Col 70 Sector,Unknown,207.125,-49.71875,-1147.84375
+Col 70 Sector HP-Q c5-4,Col 70 Sector,Unknown,820.8125,-626.625,-1360.5
+Col 70 Sector HQ-W b31-0,Col 70 Sector,Unknown,450.90625,-419.875,-906.4375
+Col 70 Sector HQ-Z b3-0,Col 70 Sector,Unknown,684.8125,-191.875,-1520.65625
+Col 70 Sector HS-R b34-0,Col 70 Sector,Unknown,276.65625,-384.4375,-863
+Col 70 Sector HT-E b42-1,Col 70 Sector,Unknown,409.3125,-250.5625,-690.59375
+Col 70 Sector HU-M b25-2,Col 70 Sector,Unknown,765.65625,-24.03125,-1050.5
+Col 70 Sector HV-W c2-0,Col 70 Sector,Unknown,669.9375,-432.34375,-1494.84375
+Col 70 Sector HW-L b37-0,Col 70 Sector,Unknown,447.15625,-427.03125,-786.34375
+Col 70 Sector HW-M b36-0,Col 70 Sector,Unknown,365.6875,-516.34375,-805.09375
+Col 70 Sector HX-N b35-0,Col 70 Sector,Unknown,797.8125,-600.1875,-842.5625
+Col 70 Sector HY-D c14-8,Col 70 Sector,Unknown,760.15625,28.125,-988.0625
+Col 70 Sector HY-H d10-2,Col 70 Sector,Unknown,239.125,-312.125,-695.0625
+Col 70 Sector HY-H d10-23,Col 70 Sector,Unknown,221.375,-269.3125,-708.75
+Col 70 Sector HY-S b33-0,Col 70 Sector,Unknown,760.03125,-442.46875,-884.5625
+Col 70 Sector HY-W b32-0,Col 70 Sector,Unknown,285.4375,-197.8125,-899.1875
+Col 70 Sector HZ-C b30-1,Col 70 Sector,Unknown,171.65625,-110.09375,-955.125
+Col 70 Sector HZ-P b35-0,Col 70 Sector,Unknown,720.59375,-379.1875,-844.8125
+Col 70 Sector IC-P b24-0,Col 70 Sector,Unknown,455.90625,36.75,-1076.0625
+Col 70 Sector ID-I d10-36,Col 70 Sector,Unknown,440.0625,-238.4375,-693.375
+Col 70 Sector ID-M a71-0,Col 70 Sector,Unknown,290.46875,-38.21875,-836.15625
+Col 70 Sector ID-R d5-24,Col 70 Sector,Unknown,95.78125,-162.8125,-1066.25
+Col 70 Sector IE-E b42-0,Col 70 Sector,Unknown,295.78125,-322.625,-699.5
+Col 70 Sector IF-H b26-0,Col 70 Sector,Unknown,416.34375,-406.5625,-1025.0625
+Col 70 Sector IF-I b41-0,Col 70 Sector,Unknown,336.03125,-84.125,-721.46875
+Col 70 Sector IG-E c12-10,Col 70 Sector,Unknown,783.375,-626.4375,-1092.34375
+Col 70 Sector IG-M c8-7,Col 70 Sector,Unknown,802.09375,-434.125,-1255.28125
+Col 70 Sector II-I d10-8,Col 70 Sector,Unknown,575.84375,-137.25,-723.125
+Col 70 Sector IJ-G b27-0,Col 70 Sector,Unknown,18.09375,-321.21875,-1023.15625
+Col 70 Sector IJ-V b33-0,Col 70 Sector,Unknown,265.09375,-178.84375,-874.125
+Col 70 Sector IJ-W b45-0,Col 70 Sector,Unknown,343.15625,-388.0625,-616.71875
+Col 70 Sector IL-N c7-5,Col 70 Sector,Unknown,699.75,-552.5,-1297.0625
+Col 70 Sector IM-K b38-0,Col 70 Sector,Unknown,461.6875,-390.53125,-769.21875
+Col 70 Sector IM-Z b18-1,Col 70 Sector,Unknown,383.90625,17.75,-1195.625
+Col 70 Sector IN-J d9-13,Col 70 Sector,Unknown,468.15625,-355.4375,-801.5625
+Col 70 Sector IN-J d9-17,Col 70 Sector,Unknown,456.0625,-362.875,-780.09375
+Col 70 Sector IN-J d9-18,Col 70 Sector,Unknown,474.59375,-397.3125,-747.53125
+Col 70 Sector IN-J d9-6,Col 70 Sector,Unknown,440.84375,-421.78125,-803.96875
+Col 70 Sector IN-J d9-7,Col 70 Sector,Unknown,460.96875,-385.4375,-806.84375
+Col 70 Sector IN-J d9-8,Col 70 Sector,Unknown,458.5,-387.625,-798.40625
+Col 70 Sector IO-E b42-0,Col 70 Sector,Unknown,391.59375,-281.28125,-690.9375
+Col 70 Sector IO-U c18-3,Col 70 Sector,Unknown,780.25,-98.375,-812.21875
+Col 70 Sector IO-U c18-8,Col 70 Sector,Unknown,787.96875,-93.5,-805.0625
+Col 70 Sector IP-B b44-1,Col 70 Sector,Unknown,339.78125,-216.5625,-662.25
+Col 70 Sector IP-B c15-6,Col 70 Sector,Unknown,411.53125,-68.0625,-971.375
+Col 70 Sector IS-J d9-18,Col 70 Sector,Unknown,604.71875,-341.28125,-745.71875
+Col 70 Sector IS-R b21-0,Col 70 Sector,Unknown,142.1875,-236.3125,-1125.1875
+Col 70 Sector IT-C b31-0,Col 70 Sector,Unknown,415.53125,40.25,-942.40625
+Col 70 Sector IT-M c9-2,Col 70 Sector,Unknown,539.28125,45.8125,-1213.34375
+Col 70 Sector IV-Z b16-0,Col 70 Sector,Unknown,898.71875,-320.21875,-1242.4375
+Col 70 Sector IW-L b37-0,Col 70 Sector,Unknown,458.78125,-441.125,-793.125
+Col 70 Sector IX-J d9-15,Col 70 Sector,Unknown,771.78125,-254.75,-783.59375
+Col 70 Sector IX-J d9-30,Col 70 Sector,Unknown,744.25,-248.3125,-798.0625
+Col 70 Sector IX-J d9-31,Col 70 Sector,Unknown,744.75,-242.15625,-807.03125
+Col 70 Sector IY-H d10-12,Col 70 Sector,Unknown,268.59375,-294.8125,-728.59375
+Col 70 Sector IY-H d10-14,Col 70 Sector,Unknown,296.25,-285.59375,-700.15625
+Col 70 Sector IY-H d10-16,Col 70 Sector,Unknown,291.9375,-325.53125,-685.8125
+Col 70 Sector IY-H d10-17,Col 70 Sector,Unknown,293.53125,-341.5625,-677.5625
+Col 70 Sector IY-H d10-21,Col 70 Sector,Unknown,265.71875,-309.21875,-668.3125
+Col 70 Sector IY-H d10-24,Col 70 Sector,Unknown,318.5625,-296,-723.6875
+Col 70 Sector IY-X c15-0,Col 70 Sector,Unknown,458.375,-482.1875,-931.6875
+Col 70 Sector IZ-D c13-2,Col 70 Sector,Unknown,248.78125,-337.1875,-1052.875
+Col 70 Sector IZ-M b37-0,Col 70 Sector,Unknown,178.375,-323.5,-802.34375
+Col 70 Sector JB-D b29-0,Col 70 Sector,Unknown,437.625,-303.8125,-982.46875
+Col 70 Sector JB-W d2-2,Col 70 Sector,Unknown,871.625,-328.6875,-1378.21875
+Col 70 Sector JC-T d4-26,Col 70 Sector,Unknown,696.25,-88.375,-1186.03125
+Col 70 Sector JE-V c17-0,Col 70 Sector,Unknown,534.46875,-315.90625,-843.21875
+Col 70 Sector JF-O b36-0,Col 70 Sector,Unknown,677.875,-382.09375,-823.125
+Col 70 Sector JG-E c12-9,Col 70 Sector,Unknown,816.4375,-626.625,-1099.875
+Col 70 Sector JG-M c8-4,Col 70 Sector,Unknown,819.0625,-428.125,-1229.84375
+Col 70 Sector JG-N c7-7,Col 70 Sector,Unknown,662.3125,-617.09375,-1302.6875
+Col 70 Sector JG-X d1-9,Col 70 Sector,Unknown,657.78125,-560.125,-1428.8125
+Col 70 Sector JH-I b14-0,Col 70 Sector,Unknown,277.71875,17.4375,-1300.84375
+Col 70 Sector JI-K b12-0,Col 70 Sector,Unknown,636.03125,-143.34375,-1340.71875
+Col 70 Sector JJ-G b27-0,Col 70 Sector,Unknown,37,-322.5625,-1020.71875
+Col 70 Sector JK-B b31-0,Col 70 Sector,Unknown,164.8125,-93.25,-937.375
+Col 70 Sector JK-B b44-0,Col 70 Sector,Unknown,329.90625,-226.875,-647.96875
+Col 70 Sector JK-C b43-0,Col 70 Sector,Unknown,247.78125,-316.375,-668.375
+Col 70 Sector JM-A b31-0,Col 70 Sector,Unknown,479.40625,-201.875,-940.78125
+Col 70 Sector JM-K b38-0,Col 70 Sector,Unknown,478.71875,-400.8125,-781.875
+Col 70 Sector JN-H b27-0,Col 70 Sector,Unknown,286.78125,-196.71875,-1018.8125
+Col 70 Sector JP-C b43-0,Col 70 Sector,Unknown,275.59375,-303.375,-679.3125
+Col 70 Sector JQ-R c19-2,Col 70 Sector,Unknown,179.5625,-330.65625,-771.84375
+Col 70 Sector JR-B b30-0,Col 70 Sector,Unknown,436.1875,-264.75,-961.90625
+Col 70 Sector JT-D c14-9,Col 70 Sector,Unknown,760.21875,8.5625,-1015.25
+Col 70 Sector JT-E b42-0,Col 70 Sector,Unknown,443.9375,-252.5625,-700.21875
+Col 70 Sector JT-F c12-7,Col 70 Sector,Unknown,425.3125,-340.09375,-1067.25
+Col 70 Sector JT-H d10-7,Col 70 Sector,Unknown,221.96875,-359.8125,-681.90625
+Col 70 Sector JT-P d6-6,Col 70 Sector,Unknown,215.34375,-24.75,-1029.90625
+Col 70 Sector JV-R c19-1,Col 70 Sector,Unknown,276.375,-269.1875,-746.65625
+Col 70 Sector JW-L b37-0,Col 70 Sector,Unknown,479.75,-439.28125,-799.96875
+Col 70 Sector JX-A b30-0,Col 70 Sector,Unknown,281.34375,-343.5625,-950.625
+Col 70 Sector JY-H d10-10,Col 70 Sector,Unknown,396.28125,-291.28125,-707.9375
+Col 70 Sector JY-H d10-9,Col 70 Sector,Unknown,391.90625,-297.53125,-716.46875
+Col 70 Sector JZ-V b32-0,Col 70 Sector,Unknown,116.65625,-299.25,-902.5
+Col 70 Sector KD-I d10-25,Col 70 Sector,Unknown,581.75,-201.78125,-711.875
+Col 70 Sector KD-J d9-10,Col 70 Sector,Unknown,261.46875,-564.125,-757.75
+Col 70 Sector KD-J d9-3,Col 70 Sector,Unknown,320.0625,-578.15625,-763.40625
+Col 70 Sector KD-R d5-21,Col 70 Sector,Unknown,294.40625,-122.40625,-1120.25
+Col 70 Sector KD-X b45-1,Col 70 Sector,Unknown,553.125,-306.65625,-618.5
+Col 70 Sector KE-R b34-0,Col 70 Sector,Unknown,754.75,-430.40625,-856.3125
+Col 70 Sector KE-T a67-0,Col 70 Sector,Unknown,207.6875,-44.78125,-883.125
+Col 70 Sector KE-W b45-0,Col 70 Sector,Unknown,345.375,-408.1875,-617.59375
+Col 70 Sector KF-L b38-0,Col 70 Sector,Unknown,149.0625,-310.4375,-782.8125
+Col 70 Sector KF-U c17-0,Col 70 Sector,Unknown,138.625,-541,-862.59375
+Col 70 Sector KI-J d9-1,Col 70 Sector,Unknown,417.75,-444.6875,-801.875
+Col 70 Sector KI-K b12-0,Col 70 Sector,Unknown,658.09375,-142.90625,-1339.125
+Col 70 Sector KJ-G d11-9,Col 70 Sector,Unknown,327.8125,-204.0625,-647.84375
+Col 70 Sector KK-L b38-0,Col 70 Sector,Unknown,179.28125,-301.1875,-781.5625
+Col 70 Sector KL-R c19-3,Col 70 Sector,Unknown,169.34375,-362.53125,-765.09375
+Col 70 Sector KL-W b31-0,Col 70 Sector,Unknown,460.46875,-431.59375,-920.21875
+Col 70 Sector KM-C b29-0,Col 70 Sector,Unknown,339.96875,-361.25,-981.25
+Col 70 Sector KM-L c8-2,Col 70 Sector,Unknown,539.4375,-601.8125,-1246.09375
+Col 70 Sector KM-U d3-17,Col 70 Sector,Unknown,762.6875,-253.8125,-1290.28125
+Col 70 Sector KM-U d3-18,Col 70 Sector,Unknown,744.0625,-260.15625,-1274.8125
+Col 70 Sector KN-R b21-0,Col 70 Sector,Unknown,154.75,-252.46875,-1143.1875
+Col 70 Sector KO-P d6-17,Col 70 Sector,Unknown,152.9375,-37.90625,-1063.1875
+Col 70 Sector KQ-J b27-1,Col 70 Sector,Unknown,754.875,22.9375,-1008.625
+Col 70 Sector KT-E b42-1,Col 70 Sector,Unknown,455.84375,-259.21875,-702.21875
+Col 70 Sector KT-N b24-1,Col 70 Sector,Unknown,221.90625,-88.40625,-1068.5625
+Col 70 Sector KT-O b36-0,Col 70 Sector,Unknown,297.1875,-320.375,-820.15625
+Col 70 Sector KT-V b46-0,Col 70 Sector,Unknown,540.9375,-284.5,-604.53125
+Col 70 Sector KU-D b42-0,Col 70 Sector,Unknown,255.8125,-362,-695.75
+Col 70 Sector KV-L b38-0,Col 70 Sector,Unknown,778.0625,-259.4375,-784.71875
+Col 70 Sector KX-I b39-0,Col 70 Sector,Unknown,458.125,-381.875,-762.21875
+Col 70 Sector KY-Q d5-11,Col 70 Sector,Unknown,153.09375,-221.90625,-1066.875
+Col 70 Sector KY-W b19-0,Col 70 Sector,Unknown,189.5,-49.75,-1180.59375
+Col 70 Sector KZ-U b46-0,Col 70 Sector,Unknown,378.5625,-361.15625,-604.9375
+Col 70 Sector KZ-V b32-0,Col 70 Sector,Unknown,137.21875,-304.90625,-899.96875
+Col 70 Sector LA-L b26-2,Col 70 Sector,Unknown,769.875,-17.5625,-1033.4375
+Col 70 Sector LC-K b38-0,Col 70 Sector,Unknown,436.8125,-443.15625,-781.9375
+Col 70 Sector LD-M b36-0,Col 70 Sector,Unknown,797.875,-603.59375,-820.3125
+Col 70 Sector LE-D b43-1,Col 70 Sector,Unknown,440.65625,-230.03125,-679.0625
+Col 70 Sector LE-V b33-0,Col 70 Sector,Unknown,280.34375,-187.25,-884.84375
+Col 70 Sector LG-Q c20-0,Col 70 Sector,Unknown,256.1875,-262.375,-741.875
+Col 70 Sector LG-Q c20-1,Col 70 Sector,Unknown,284.25,-244.15625,-728.875
+Col 70 Sector LH-K b38-0,Col 70 Sector,Unknown,489.375,-405.46875,-775.96875
+Col 70 Sector LI-Z b30-0,Col 70 Sector,Unknown,280.625,-324.3125,-941.25
+Col 70 Sector LJ-G d11-0,Col 70 Sector,Unknown,335.5,-252.53125,-650.875
+Col 70 Sector LJ-G d11-17,Col 70 Sector,Unknown,397.78125,-228.875,-652.34375
+Col 70 Sector LJ-G d11-24,Col 70 Sector,Unknown,343.6875,-242.625,-658.125
+Col 70 Sector LJ-G d11-40,Col 70 Sector,Unknown,378.03125,-209.46875,-650.5
+Col 70 Sector LJ-W c16-1,Col 70 Sector,Unknown,497.59375,-462.6875,-899.375
+Col 70 Sector LK-C b43-0,Col 70 Sector,Unknown,277.65625,-319.5,-685
+Col 70 Sector LL-R c19-0,Col 70 Sector,Unknown,194.71875,-375.15625,-770.84375
+Col 70 Sector LO-P d6-1,Col 70 Sector,Unknown,207.25,-82.46875,-1050.21875
+Col 70 Sector LP-B b44-0,Col 70 Sector,Unknown,399.3125,-223.84375,-663.5
+Col 70 Sector LR-M b36-0,Col 70 Sector,Unknown,411.84375,-544.5,-811.5625
+Col 70 Sector LR-S c5-1,Col 70 Sector,Unknown,834.96875,-212.65625,-1356.25
+Col 70 Sector LS-W a65-0,Col 70 Sector,Unknown,302.6875,-43.6875,-895.28125
+Col 70 Sector LT-O b36-0,Col 70 Sector,Unknown,319.6875,-319.3125,-824.15625
+Col 70 Sector LU-K b39-1,Col 70 Sector,Unknown,357.03125,-180.25,-748.4375
+Col 70 Sector LU-S c19-5,Col 70 Sector,Unknown,760,-96.8125,-768.375
+Col 70 Sector LV-J c10-1,Col 70 Sector,Unknown,28.15625,-202.96875,-1147.71875
+Col 70 Sector LV-O c6-2,Col 70 Sector,Unknown,818.28125,-626.34375,-1308.8125
+Col 70 Sector LV-O c6-6,Col 70 Sector,Unknown,822.8125,-626.625,-1333.1875
+Col 70 Sector LV-R c19-1,Col 70 Sector,Unknown,338.15625,-303.53125,-747.5
+Col 70 Sector LV-Z b44-1,Col 70 Sector,Unknown,325.46875,-223.5625,-635.78125
+Col 70 Sector LW-U b32-0,Col 70 Sector,Unknown,438.34375,-419.625,-902.25
+Col 70 Sector LW-V d2-4,Col 70 Sector,Unknown,831.25,-394.46875,-1316.375
+Col 70 Sector LW-V d2-5,Col 70 Sector,Unknown,873.15625,-380.71875,-1360.28125
+Col 70 Sector LW-V d2-7,Col 70 Sector,Unknown,854.09375,-389.53125,-1316.125
+Col 70 Sector LW-X b4-0,Col 70 Sector,Unknown,680.03125,-200.6875,-1500.15625
+Col 70 Sector LW-Z b18-0,Col 70 Sector,Unknown,515.9375,60.625,-1200.875
+Col 70 Sector LX-K d8-10,Col 70 Sector,Unknown,733.84375,-558.78125,-858.65625
+Col 70 Sector LX-K d8-2,Col 70 Sector,Unknown,703.09375,-542.125,-884.46875
+Col 70 Sector LX-K d8-3,Col 70 Sector,Unknown,687.34375,-546.5,-898.46875
+Col 70 Sector LX-K d8-9,Col 70 Sector,Unknown,721.28125,-552.875,-865.96875
+Col 70 Sector LX-S d4-15,Col 70 Sector,Unknown,731.90625,-174.15625,-1189.15625
+Col 70 Sector LX-S d4-9,Col 70 Sector,Unknown,691.5625,-151.875,-1187.46875
+Col 70 Sector LY-P b22-0,Col 70 Sector,Unknown,116.84375,-229.4375,-1118.84375
+Col 70 Sector LY-Q d5-12,Col 70 Sector,Unknown,180.71875,-225.25,-1072.3125
+Col 70 Sector LZ-A b32-1,Col 70 Sector,Unknown,400.46875,42.65625,-914.46875
+Col 70 Sector LZ-D c13-0,Col 70 Sector,Unknown,335.40625,-342.3125,-1062.71875
+Col 70 Sector LZ-V b32-0,Col 70 Sector,Unknown,155.0625,-293.03125,-894.28125
+Col 70 Sector MA-B c15-0,Col 70 Sector,Unknown,304.1875,-216.84375,-953.90625
+Col 70 Sector MA-K c10-0,Col 70 Sector,Unknown,139.90625,-181.21875,-1183.09375
+Col 70 Sector MA-U c17-0,Col 70 Sector,Unknown,159.375,-573.1875,-850.90625
+Col 70 Sector MA-U c17-1,Col 70 Sector,Unknown,136.125,-559.5625,-851.3125
+Col 70 Sector MC-L b37-0,Col 70 Sector,Unknown,375.3125,-522.625,-800.34375
+Col 70 Sector MD-I d10-25,Col 70 Sector,Unknown,736.8125,-240.28125,-667.4375
+Col 70 Sector MD-Y b31-0,Col 70 Sector,Unknown,343.0625,-251.40625,-910.90625
+Col 70 Sector ME-G d11-11,Col 70 Sector,Unknown,329.9375,-307.9375,-615
+Col 70 Sector ME-G d11-12,Col 70 Sector,Unknown,333.8125,-310.125,-618.125
+Col 70 Sector ME-G d11-13,Col 70 Sector,Unknown,326.34375,-324.21875,-624.28125
+Col 70 Sector ME-G d11-15,Col 70 Sector,Unknown,334.46875,-321.53125,-639.4375
+Col 70 Sector ME-G d11-16,Col 70 Sector,Unknown,334.03125,-310.96875,-625.90625
+Col 70 Sector ME-G d11-17,Col 70 Sector,Unknown,323.75,-315.03125,-641.75
+Col 70 Sector ME-G d11-26,Col 70 Sector,Unknown,321.9375,-311.65625,-632.90625
+Col 70 Sector ME-G d11-5,Col 70 Sector,Unknown,321.6875,-337.78125,-653.90625
+Col 70 Sector ME-W c16-0,Col 70 Sector,Unknown,460.96875,-502.1875,-904.90625
+Col 70 Sector MF-C b43-0,Col 70 Sector,Unknown,273.0625,-335.1875,-672.75
+Col 70 Sector MF-C c14-0,Col 70 Sector,Unknown,231.4375,-331.21875,-1018.5
+Col 70 Sector MG-F b27-0,Col 70 Sector,Unknown,377.90625,-439.5,-1019.6875
+Col 70 Sector MG-Q c20-0,Col 70 Sector,Unknown,299.1875,-264.3125,-741.34375
+Col 70 Sector MJ-G d11-37,Col 70 Sector,Unknown,433.1875,-200.21875,-628.21875
+Col 70 Sector MJ-G d11-8,Col 70 Sector,Unknown,436.03125,-213.84375,-641.21875
+Col 70 Sector MJ-G d11-9,Col 70 Sector,Unknown,441.21875,-215.40625,-656.09375
+Col 70 Sector MJ-K a72-1,Col 70 Sector,Unknown,290.90625,-38.53125,-834.625
+Col 70 Sector MK-E a48-0,Col 70 Sector,Unknown,307.84375,-34.90625,-1092.875
+Col 70 Sector MK-Z b32-1,Col 70 Sector,Unknown,390.6875,55.0625,-886.90625
+Col 70 Sector MM-L c8-4,Col 70 Sector,Unknown,650.1875,-596.8125,-1255.8125
+Col 70 Sector MM-U c3-7,Col 70 Sector,Unknown,480,-568.90625,-1430.0625
+Col 70 Sector MN-O a56-1,Col 70 Sector,Unknown,214.8125,-33.1875,-999.84375
+Col 70 Sector MP-D b42-0,Col 70 Sector,Unknown,267.09375,-374.78125,-700.25
+Col 70 Sector MP-J b40-0,Col 70 Sector,Unknown,420.28125,-108.9375,-738
+Col 70 Sector MP-P b35-0,Col 70 Sector,Unknown,740.125,-420.34375,-841.40625
+Col 70 Sector MR-K c9-1,Col 70 Sector,Unknown,880.875,-413.90625,-1196.625
+Col 70 Sector MS-A b30-0,Col 70 Sector,Unknown,296.15625,-364.5,-957.5
+Col 70 Sector MS-C a49-1,Col 70 Sector,Unknown,785.78125,-34.375,-1084.53125
+Col 70 Sector MT-D a62-1,Col 70 Sector,Unknown,209.25,-38.6875,-942.75
+Col 70 Sector MT-D b43-2,Col 70 Sector,Unknown,575.5625,-184.75,-681.375
+Col 70 Sector MT-P d6-17,Col 70 Sector,Unknown,465.375,-3.59375,-1010.71875
+Col 70 Sector MT-P d6-21,Col 70 Sector,Unknown,477.46875,-2.65625,-1003.15625
+Col 70 Sector MT-P d6-36,Col 70 Sector,Unknown,436.4375,40.4375,-994.375
+Col 70 Sector MU-C b43-0,Col 70 Sector,Unknown,380.40625,-270.75,-667.875
+Col 70 Sector MU-M b37-0,Col 70 Sector,Unknown,220.90625,-345,-800.59375
+Col 70 Sector MU-S c19-0,Col 70 Sector,Unknown,779.9375,-98.0625,-765.5625
+Col 70 Sector MU-U c17-0,Col 70 Sector,Unknown,455.5625,-422.46875,-862.25
+Col 70 Sector MU-U c17-2,Col 70 Sector,Unknown,464.25,-419.375,-860.71875
+Col 70 Sector MV-A b44-0,Col 70 Sector,Unknown,267,-301.40625,-655.09375
+Col 70 Sector MV-Z b31-0,Col 70 Sector,Unknown,180.3125,-80.28125,-919.65625
+Col 70 Sector MW-V d2-7,Col 70 Sector,Unknown,898.96875,-374.3125,-1356.625
+Col 70 Sector MX-K d8-2,Col 70 Sector,Unknown,753.3125,-578.15625,-862.25
+Col 70 Sector MZ-V b32-0,Col 70 Sector,Unknown,188.96875,-299.21875,-904.03125
+Col 70 Sector NA-C b43-0,Col 70 Sector,Unknown,238.96875,-364.15625,-679.9375
+Col 70 Sector NB-H b41-0,Col 70 Sector,Unknown,201.3125,-202.5625,-719
+Col 70 Sector NB-Y b17-0,Col 70 Sector,Unknown,931.1875,-305.75,-1212.5
+Col 70 Sector ND-X a51-0,Col 70 Sector,Unknown,143.34375,-36.5625,-1047.84375
+Col 70 Sector NE-G d11-10,Col 70 Sector,Unknown,351.8125,-324.0625,-650.78125
+Col 70 Sector NE-G d11-11,Col 70 Sector,Unknown,372.65625,-325.90625,-612.90625
+Col 70 Sector NE-G d11-13,Col 70 Sector,Unknown,352.28125,-325.15625,-618.71875
+Col 70 Sector NE-G d11-14,Col 70 Sector,Unknown,357.6875,-332.90625,-626.15625
+Col 70 Sector NE-G d11-26,Col 70 Sector,Unknown,369.75,-317.5625,-647.8125
+Col 70 Sector NE-G d11-27,Col 70 Sector,Unknown,359.65625,-323.90625,-648.5625
+Col 70 Sector NE-G d11-28,Col 70 Sector,Unknown,358.0625,-278.125,-616.59375
+Col 70 Sector NE-G d11-3,Col 70 Sector,Unknown,369.59375,-321.03125,-603.71875
+Col 70 Sector NF-C c14-2,Col 70 Sector,Unknown,258.4375,-307.25,-993.78125
+Col 70 Sector NF-L b38-0,Col 70 Sector,Unknown,195.1875,-322.125,-779.25
+Col 70 Sector NF-T c18-1,Col 70 Sector,Unknown,417.59375,-376.15625,-816.28125
+Col 70 Sector NF-U b33-0,Col 70 Sector,Unknown,118.46875,-300.0625,-882.6875
+Col 70 Sector NG-L a44-0,Col 70 Sector,Unknown,199.34375,-38.4375,-1132.96875
+Col 70 Sector NG-W b31-0,Col 70 Sector,Unknown,492.03125,-463.03125,-908
+Col 70 Sector NH-T b33-0,Col 70 Sector,Unknown,435.21875,-400.375,-879.875
+Col 70 Sector NI-H b40-0,Col 70 Sector,Unknown,490.53125,-350.28125,-725.5625
+Col 70 Sector NJ-H d10-6,Col 70 Sector,Unknown,252.0625,-569.625,-725.03125
+Col 70 Sector NJ-O b36-0,Col 70 Sector,Unknown,278,-364.25,-820.21875
+Col 70 Sector NL-J b27-2,Col 70 Sector,Unknown,761.4375,-2.65625,-1024.9375
+Col 70 Sector NL-R c19-0,Col 70 Sector,Unknown,258.03125,-382.34375,-783.71875
+Col 70 Sector NM-C b29-0,Col 70 Sector,Unknown,395.15625,-362.375,-972.75
+Col 70 Sector NM-V d2-0,Col 70 Sector,Unknown,708.5625,-564,-1381
+Col 70 Sector NM-V d2-1,Col 70 Sector,Unknown,691.78125,-564.21875,-1382.3125
+Col 70 Sector NM-V d2-4,Col 70 Sector,Unknown,668.375,-579.21875,-1337.625
+Col 70 Sector NM-V d2-6,Col 70 Sector,Unknown,691.71875,-559.28125,-1327.75
+Col 70 Sector NP-B b44-0,Col 70 Sector,Unknown,440.4375,-223.40625,-661.28125
+Col 70 Sector NP-C b43-0,Col 70 Sector,Unknown,373.1875,-287.375,-668.625
+Col 70 Sector NP-U c17-2,Col 70 Sector,Unknown,447.1875,-446.5,-859.5
+Col 70 Sector NQ-Z b44-1,Col 70 Sector,Unknown,330.375,-231.0625,-630.46875
+Col 70 Sector NS-A b30-0,Col 70 Sector,Unknown,316.625,-360.625,-964.1875
+Col 70 Sector NT-H d10-11,Col 70 Sector,Unknown,499.75,-359.9375,-732.09375
+Col 70 Sector NT-P d6-21,Col 70 Sector,Unknown,497.40625,25.4375,-1030.09375
+Col 70 Sector NU-C c14-4,Col 70 Sector,Unknown,508.1875,-210.0625,-1019.96875
+Col 70 Sector NV-I b12-0,Col 70 Sector,Unknown,915.6875,-302.03125,-1344.25
+Col 70 Sector NW-D b28-0,Col 70 Sector,Unknown,397.15625,-401.125,-999.21875
+Col 70 Sector NY-I d9-1,Col 70 Sector,Unknown,354.71875,-587.90625,-782.8125
+Col 70 Sector NZ-U c17-0,Col 70 Sector,Unknown,611.3125,-358.65625,-833.34375
+Col 70 Sector OA-B b44-0,Col 70 Sector,Unknown,337.46875,-278.15625,-651.03125
+Col 70 Sector OA-J b40-0,Col 70 Sector,Unknown,346.8125,-179.3125,-730.875
+Col 70 Sector OA-K b26-0,Col 70 Sector,Unknown,109.90625,-105.9375,-1033.34375
+Col 70 Sector OB-P b9-0,Col 70 Sector,Unknown,856.34375,-199.875,-1401.375
+Col 70 Sector OB-P c21-10,Col 70 Sector,Unknown,471.03125,-143.84375,-698.25
+Col 70 Sector OB-Q c20-1,Col 70 Sector,Unknown,331.25,-284.21875,-708.71875
+Col 70 Sector OB-Q c20-4,Col 70 Sector,Unknown,325.375,-290.59375,-722.71875
+Col 70 Sector OB-Y b17-0,Col 70 Sector,Unknown,946.84375,-318.25,-1216.46875
+Col 70 Sector OC-K b38-0,Col 70 Sector,Unknown,496.5625,-429.59375,-765.5
+Col 70 Sector OC-Y b19-0,Col 70 Sector,Unknown,509.375,71.40625,-1171.5
+Col 70 Sector OD-R d5-12,Col 70 Sector,Unknown,578.21875,-143.25,-1098.28125
+Col 70 Sector OE-H b27-0,Col 70 Sector,Unknown,819.25,-242.6875,-1024.125
+Col 70 Sector OF-T c18-0,Col 70 Sector,Unknown,460.09375,-381.34375,-821.25
+Col 70 Sector OF-T c18-3,Col 70 Sector,Unknown,488.125,-360,-810.40625
+Col 70 Sector OF-T c18-4,Col 70 Sector,Unknown,491.5,-360.78125,-815.0625
+Col 70 Sector OG-A c15-0,Col 70 Sector,Unknown,73.0625,-353.28125,-957.6875
+Col 70 Sector OG-Y b32-1,Col 70 Sector,Unknown,178.53125,-60.3125,-901.59375
+Col 70 Sector OH-V d2-10,Col 70 Sector,Unknown,598.46875,-610.21875,-1311.28125
+Col 70 Sector OH-Y a23-0,Col 70 Sector,Unknown,591.71875,-33.90625,-1352.4375
+Col 70 Sector OI-J d9-15,Col 70 Sector,Unknown,762.5625,-468.4375,-763.8125
+Col 70 Sector OJ-H d10-10,Col 70 Sector,Unknown,265.34375,-569.03125,-714.125
+Col 70 Sector OJ-W b45-0,Col 70 Sector,Unknown,473.03125,-390.125,-622.71875
+Col 70 Sector ON-I b39-0,Col 70 Sector,Unknown,473.3125,-416.5625,-751.65625
+Col 70 Sector OP-L b38-0,Col 70 Sector,Unknown,295.40625,-273.3125,-772.90625
+Col 70 Sector OP-S c19-4,Col 70 Sector,Unknown,781.6875,-108.625,-783.71875
+Col 70 Sector OP-S c19-5,Col 70 Sector,Unknown,785.28125,-105.9375,-779.75
+Col 70 Sector OP-S c19-9,Col 70 Sector,Unknown,789.71875,-113.15625,-765.75
+Col 70 Sector OQ-Q c20-1,Col 70 Sector,Unknown,566.78125,-157.46875,-712.625
+Col 70 Sector OR-A a50-0,Col 70 Sector,Unknown,227.78125,-43.46875,-1074.375
+Col 70 Sector OR-D b28-0,Col 70 Sector,Unknown,377.8125,-419.8125,-999.90625
+Col 70 Sector OS-I b39-0,Col 70 Sector,Unknown,495.375,-402.0625,-760.375
+Col 70 Sector OT-H d10-4,Col 70 Sector,Unknown,651.1875,-347.46875,-734.3125
+Col 70 Sector OT-Q d5-12,Col 70 Sector,Unknown,262.4375,-275.4375,-1074.3125
+Col 70 Sector OV-Z b44-0,Col 70 Sector,Unknown,388.46875,-216.40625,-625.09375
+Col 70 Sector OY-L b36-0,Col 70 Sector,Unknown,820.15625,-619.75,-821.125
+Col 70 Sector OZ-F d11-1,Col 70 Sector,Unknown,305,-369.875,-629.03125
+Col 70 Sector OZ-F d11-11,Col 70 Sector,Unknown,316.5,-345.59375,-630.53125
+Col 70 Sector OZ-F d11-2,Col 70 Sector,Unknown,293,-367.6875,-639.75
+Col 70 Sector OZ-F d11-6,Col 70 Sector,Unknown,289.9375,-367.78125,-639.5
+Col 70 Sector OZ-F d11-7,Col 70 Sector,Unknown,270.3125,-376.375,-654.90625
+Col 70 Sector OZ-M b24-0,Col 70 Sector,Unknown,143.65625,-178.875,-1084.0625
+Col 70 Sector OZ-M b37-0,Col 70 Sector,Unknown,298.75,-324.78125,-802.8125
+Col 70 Sector OZ-V b32-0,Col 70 Sector,Unknown,216.5,-293.15625,-899.03125
+Col 70 Sector OZ-V c16-1,Col 70 Sector,Unknown,459.5625,-518.90625,-889.84375
+Col 70 Sector PA-K b39-0,Col 70 Sector,Unknown,275.3125,-260.84375,-760.28125
+Col 70 Sector PA-T c18-0,Col 70 Sector,Unknown,418.5,-423.8125,-823.0625
+Col 70 Sector PA-T c18-1,Col 70 Sector,Unknown,432.59375,-421.84375,-809.78125
+Col 70 Sector PA-T c18-2,Col 70 Sector,Unknown,433.1875,-399.5,-799.0625
+Col 70 Sector PA-T c18-3,Col 70 Sector,Unknown,420.5,-407.5625,-814.625
+Col 70 Sector PA-V b20-0,Col 70 Sector,Unknown,679.90625,-81.1875,-1162.375
+Col 70 Sector PB-N c7-8,Col 70 Sector,Unknown,819.3125,-625.6875,-1281.78125
+Col 70 Sector PB-Q c20-2,Col 70 Sector,Unknown,353.6875,-273.125,-718.03125
+Col 70 Sector PC-J b39-0,Col 70 Sector,Unknown,608.84375,-357.09375,-760.53125
+Col 70 Sector PC-P b37-2,Col 70 Sector,Unknown,756.5,-97.78125,-797.5625
+Col 70 Sector PC-T b33-0,Col 70 Sector,Unknown,436.0625,-423.0625,-879.59375
+Col 70 Sector PC-U d3-6,Col 70 Sector,Unknown,817.34375,-401.5625,-1296.09375
+Col 70 Sector PC-U d3-7,Col 70 Sector,Unknown,825.71875,-384.625,-1303.21875
+Col 70 Sector PC-W b5-0,Col 70 Sector,Unknown,678.78125,-200.40625,-1483.53125
+Col 70 Sector PE-P d6-14,Col 70 Sector,Unknown,208.875,-244.75,-1055.65625
+Col 70 Sector PE-V b20-0,Col 70 Sector,Unknown,197.03125,-59.03125,-1163.5625
+Col 70 Sector PF-C c14-1,Col 70 Sector,Unknown,338.25,-340.6875,-1019.09375
+Col 70 Sector PF-K b39-0,Col 70 Sector,Unknown,316.65625,-241.625,-761.375
+Col 70 Sector PG-M b37-0,Col 70 Sector,Unknown,679.78125,-403.375,-786.875
+Col 70 Sector PH-B b30-0,Col 70 Sector,Unknown,475.65625,-295.15625,-957.125
+Col 70 Sector PH-E b15-0,Col 70 Sector,Unknown,880.625,-224.71875,-1272.78125
+Col 70 Sector PH-H a60-0,Col 70 Sector,Unknown,315.375,-43.25,-964.59375
+Col 70 Sector PH-V d2-2,Col 70 Sector,Unknown,668.40625,-621.1875,-1379.0625
+Col 70 Sector PH-V d2-3,Col 70 Sector,Unknown,658.96875,-638.125,-1377.78125
+Col 70 Sector PH-V d2-8,Col 70 Sector,Unknown,658.9375,-594.96875,-1359.125
+Col 70 Sector PK-N b11-0,Col 70 Sector,Unknown,600.15625,-4.84375,-1361.6875
+Col 70 Sector PN-Y b44-0,Col 70 Sector,Unknown,645.40625,-352.65625,-637.65625
+Col 70 Sector PP-E b28-0,Col 70 Sector,Unknown,79.6875,-323.71875,-1004.90625
+Col 70 Sector PQ-A b44-0,Col 70 Sector,Unknown,277.09375,-322.875,-663.25
+Col 70 Sector PQ-H b41-2,Col 70 Sector,Unknown,358.8125,-138.28125,-720.125
+Col 70 Sector PQ-Z c15-5,Col 70 Sector,Unknown,428.09375,-131.5,-933.90625
+Col 70 Sector PR-D b28-0,Col 70 Sector,Unknown,399.5,-424.1875,-1001.65625
+Col 70 Sector PR-O c21-6,Col 70 Sector,Unknown,350,-215.125,-683.125
+Col 70 Sector PR-T c4-1,Col 70 Sector,Unknown,843.0625,-354.28125,-1386.71875
+Col 70 Sector PS-J c9-6,Col 70 Sector,Unknown,611.8125,-623.15625,-1212.625
+Col 70 Sector PS-Q b35-0,Col 70 Sector,Unknown,531.90625,-302.84375,-827.25
+Col 70 Sector PU-M b37-0,Col 70 Sector,Unknown,280.375,-342.875,-802.46875
+Col 70 Sector PU-T c18-3,Col 70 Sector,Unknown,759.59375,-231.0625,-793.4375
+Col 70 Sector PV-A c15-3,Col 70 Sector,Unknown,345.65625,-246.875,-951.46875
+Col 70 Sector PV-R c19-0,Col 70 Sector,Unknown,507.28125,-279.03125,-755.96875
+Col 70 Sector PV-Z b44-1,Col 70 Sector,Unknown,399.09375,-224.90625,-639.03125
+Col 70 Sector PW-G a74-0,Col 70 Sector,Unknown,495.4375,-42.71875,-812.0625
+Col 70 Sector PW-V b31-0,Col 70 Sector,Unknown,451.25,-503.53125,-924.625
+Col 70 Sector PX-K b37-0,Col 70 Sector,Unknown,399.3125,-540.03125,-802.375
+Col 70 Sector PY-Q d5-16,Col 70 Sector,Unknown,507.0625,-247.53125,-1099.5625
+Col 70 Sector PZ-F d11-19,Col 70 Sector,Unknown,341.21875,-347.53125,-607.9375
+Col 70 Sector PZ-F d11-9,Col 70 Sector,Unknown,344.25,-410.40625,-613.875
+Col 70 Sector PZ-O d6-11,Col 70 Sector,Unknown,72.71875,-313.78125,-1019.5625
+Col 70 Sector PZ-O d6-2,Col 70 Sector,Unknown,51.34375,-279,-1031.6875
+Col 70 Sector PZ-O d6-3,Col 70 Sector,Unknown,53.4375,-321,-1017.875
+Col 70 Sector PZ-O d6-6,Col 70 Sector,Unknown,23.5,-286.96875,-1041.09375
+Col 70 Sector PZ-X b18-0,Col 70 Sector,Unknown,733.59375,-127.75,-1189.21875
+Col 70 Sector QA-B b44-0,Col 70 Sector,Unknown,375.40625,-281.34375,-663.625
+Col 70 Sector QA-B b44-1,Col 70 Sector,Unknown,375.71875,-277.84375,-654.5625
+Col 70 Sector QA-L b38-0,Col 70 Sector,Unknown,218.78125,-340.4375,-782.6875
+Col 70 Sector QA-T c18-0,Col 70 Sector,Unknown,459.65625,-419.25,-822.21875
+Col 70 Sector QA-T c18-1,Col 70 Sector,Unknown,463.15625,-416.0625,-823.1875
+Col 70 Sector QC-T b33-0,Col 70 Sector,Unknown,457.1875,-406.9375,-867.84375
+Col 70 Sector QD-H b27-0,Col 70 Sector,Unknown,338.0625,-242.34375,-1020.6875
+Col 70 Sector QD-R d5-3,Col 70 Sector,Unknown,768.65625,-112.875,-1144.28125
+Col 70 Sector QE-L b26-1,Col 70 Sector,Unknown,377.21875,-0.4375,-1039.59375
+Col 70 Sector QE-P d6-6,Col 70 Sector,Unknown,310.03125,-227.59375,-1037.5625
+Col 70 Sector QF-C c14-0,Col 70 Sector,Unknown,377.625,-342.75,-1021
+Col 70 Sector QG-Q c20-4,Col 70 Sector,Unknown,475.34375,-258.15625,-722.15625
+Col 70 Sector QG-Y b32-0,Col 70 Sector,Unknown,216.4375,-60.84375,-904.6875
+Col 70 Sector QG-Z b44-1,Col 70 Sector,Unknown,314.625,-279.4375,-626.9375
+Col 70 Sector QH-U c3-1,Col 70 Sector,Unknown,539.125,-603.4375,-1428.46875
+Col 70 Sector QH-W b18-0,Col 70 Sector,Unknown,900.09375,-306.875,-1204.1875
+Col 70 Sector QI-J b38-0,Col 70 Sector,Unknown,381.875,-507.8125,-775.40625
+Col 70 Sector QJ-G b27-0,Col 70 Sector,Unknown,182.53125,-318.9375,-1009.40625
+Col 70 Sector QJ-I a32-0,Col 70 Sector,Unknown,245.8125,-34.21875,-1263.09375
+Col 70 Sector QJ-Q e5-17,Col 70 Sector,Unknown,299.4375,-167.25,-671.9375
+Col 70 Sector QL-H c12-4,Col 70 Sector,Unknown,375.8125,19.125,-1090.5
+Col 70 Sector QL-Z b44-1,Col 70 Sector,Unknown,346.6875,-258.03125,-635.65625
+Col 70 Sector QM-D b28-0,Col 70 Sector,Unknown,378,-442.65625,-999.71875
+Col 70 Sector QN-R b34-0,Col 70 Sector,Unknown,431.71875,-403.34375,-849.03125
+Col 70 Sector QP-E b28-0,Col 70 Sector,Unknown,99.1875,-322,-1001.25
+Col 70 Sector QR-K b38-0,Col 70 Sector,Unknown,658.09375,-384.09375,-780.875
+Col 70 Sector QR-X b4-0,Col 70 Sector,Unknown,740.3125,-221.125,-1501.4375
+Col 70 Sector QS-A b30-0,Col 70 Sector,Unknown,376.40625,-361.09375,-963.46875
+Col 70 Sector QU-N d7-32,Col 70 Sector,Unknown,304.15625,-34.375,-935.90625
+Col 70 Sector QU-T b34-0,Col 70 Sector,Unknown,375.90625,-162.28125,-863.59375
+Col 70 Sector QV-A b44-0,Col 70 Sector,Unknown,339.6875,-303.21875,-663.3125
+Col 70 Sector QV-A b44-1,Col 70 Sector,Unknown,339.34375,-304.3125,-661.1875
+Col 70 Sector QV-B b43-0,Col 70 Sector,Unknown,258.3125,-383.46875,-684.5
+Col 70 Sector QW-J b39-0,Col 70 Sector,Unknown,792.5,-266.5625,-758.84375
+Col 70 Sector QY-Q d5-20,Col 70 Sector,Unknown,577.4375,-242.8125,-1072.4375
+Col 70 Sector QZ-O a42-0,Col 70 Sector,Unknown,325.0625,-33.15625,-1153.0625
+Col 70 Sector RA-K b39-0,Col 70 Sector,Unknown,315.78125,-261.15625,-764.5625
+Col 70 Sector RA-W a38-0,Col 70 Sector,Unknown,236.5,-33.96875,-1192.15625
+Col 70 Sector RF-C c14-1,Col 70 Sector,Unknown,417.34375,-340.78125,-1017.84375
+Col 70 Sector RF-U c17-1,Col 70 Sector,Unknown,426.5,-540.96875,-840.1875
+Col 70 Sector RG-A b44-0,Col 70 Sector,Unknown,246.09375,-363.21875,-649.46875
+Col 70 Sector RG-H b41-1,Col 70 Sector,Unknown,333.625,-167.90625,-710.9375
+Col 70 Sector RG-L a44-0,Col 70 Sector,Unknown,237.65625,-43.03125,-1132.5
+Col 70 Sector RH-G b29-1,Col 70 Sector,Unknown,757.40625,43.15625,-974.34375
+Col 70 Sector RH-N b10-0,Col 70 Sector,Unknown,839.625,-199.90625,-1380.28125
+Col 70 Sector RI-W b20-0,Col 70 Sector,Unknown,493.09375,59.125,-1148.75
+Col 70 Sector RJ-G b27-0,Col 70 Sector,Unknown,199.28125,-320.65625,-1021.09375
+Col 70 Sector RJ-O b36-0,Col 70 Sector,Unknown,360.625,-364.3125,-822.28125
+Col 70 Sector RK-M b37-0,Col 70 Sector,Unknown,243.75,-369.9375,-789.09375
+Col 70 Sector RL-A b44-0,Col 70 Sector,Unknown,286.9375,-338,-647.09375
+Col 70 Sector RL-I c11-4,Col 70 Sector,Unknown,272.6875,-110.625,-1131.8125
+Col 70 Sector RL-S b34-0,Col 70 Sector,Unknown,118.4375,-304.8125,-860.5
+Col 70 Sector RM-L c8-0,Col 70 Sector,Unknown,820.1875,-624.90625,-1259.9375
+Col 70 Sector RM-W b33-0,Col 70 Sector,Unknown,172.46875,-50.8125,-875.15625
+Col 70 Sector RN-H b40-0,Col 70 Sector,Unknown,607.5,-326.90625,-727
+Col 70 Sector RN-R b34-0,Col 70 Sector,Unknown,435.125,-399.28125,-863.21875
+Col 70 Sector RN-S d4-1,Col 70 Sector,Unknown,827.875,-331.3125,-1212.9375
+Col 70 Sector RN-S d4-14,Col 70 Sector,Unknown,854.53125,-316.71875,-1216.84375
+Col 70 Sector RN-S d4-5,Col 70 Sector,Unknown,875.3125,-304.53125,-1210.90625
+Col 70 Sector RO-Z a36-2,Col 70 Sector,Unknown,315.75,-34.0625,-1213.625
+Col 70 Sector RP-E b28-0,Col 70 Sector,Unknown,118.78125,-322,-1002.21875
+Col 70 Sector RP-N d7-6,Col 70 Sector,Unknown,235.03125,-161.59375,-980
+Col 70 Sector RR-G c12-3,Col 70 Sector,Unknown,118.4375,-115,-1065.0625
+Col 70 Sector RR-X b4-0,Col 70 Sector,Unknown,764.03125,-211.8125,-1490.9375
+Col 70 Sector RS-J b25-0,Col 70 Sector,Unknown,315.3125,-341.40625,-1059.6875
+Col 70 Sector RS-Q c6-2,Col 70 Sector,Unknown,816.625,-231.375,-1343.84375
+Col 70 Sector RS-S c4-8,Col 70 Sector,Unknown,497.5625,-577.21875,-1420.25
+Col 70 Sector RT-F b41-0,Col 70 Sector,Unknown,520.90625,-344.65625,-723.0625
+Col 70 Sector RV-I b40-1,Col 70 Sector,Unknown,371.65625,-200.40625,-741.5625
+Col 70 Sector RV-S c18-2,Col 70 Sector,Unknown,453.15625,-429.15625,-823.40625
+Col 70 Sector RX-B b29-0,Col 70 Sector,Unknown,357.21875,-420.5625,-981.59375
+Col 70 Sector RX-S c4-5,Col 70 Sector,Unknown,595.9375,-524.65625,-1389.78125
+Col 70 Sector RX-V b5-0,Col 70 Sector,Unknown,677.3125,-215.625,-1480.875
+Col 70 Sector RZ-O d6-1,Col 70 Sector,Unknown,216.09375,-319.90625,-1021.90625
+Col 70 Sector RZ-O d6-14,Col 70 Sector,Unknown,240.15625,-327.375,-1036.84375
+Col 70 Sector RZ-O d6-5,Col 70 Sector,Unknown,217.8125,-305.75,-991.53125
+Col 70 Sector SA-C c14-0,Col 70 Sector,Unknown,406.90625,-374.21875,-988.9375
+Col 70 Sector SA-L b38-0,Col 70 Sector,Unknown,266.21875,-330.375,-783.96875
+Col 70 Sector SB-Z b44-0,Col 70 Sector,Unknown,312.09375,-299.625,-625.1875
+Col 70 Sector SC-B c1-0,Col 70 Sector,Unknown,715.6875,-297.71875,-1550.1875
+Col 70 Sector SC-J b39-0,Col 70 Sector,Unknown,655.6875,-362.9375,-760.71875
+Col 70 Sector SC-O c21-4,Col 70 Sector,Unknown,228.84375,-340.125,-669.9375
+Col 70 Sector SC-S c5-1,Col 70 Sector,Unknown,887.875,-318.25,-1378.59375
+Col 70 Sector SD-Z b30-0,Col 70 Sector,Unknown,378.0625,-342.4375,-943.4375
+Col 70 Sector SG-H b41-0,Col 70 Sector,Unknown,352.71875,-167.625,-708.3125
+Col 70 Sector SG-T b21-1,Col 70 Sector,Unknown,657.5625,-84.90625,-1142
+Col 70 Sector SG-Z b44-0,Col 70 Sector,Unknown,340.375,-275.25,-625.46875
+Col 70 Sector SI-R b34-0,Col 70 Sector,Unknown,417.375,-421.125,-860
+Col 70 Sector SK-D c13-1,Col 70 Sector,Unknown,377.25,-459.90625,-1061.5625
+Col 70 Sector SK-L b25-0,Col 70 Sector,Unknown,175.25,-163.5,-1047.84375
+Col 70 Sector SK-M d8-10,Col 70 Sector,Unknown,429.15625,92.3125,-904.4375
+Col 70 Sector SL-A b44-0,Col 70 Sector,Unknown,296.875,-341.125,-661.65625
+Col 70 Sector SL-R c19-0,Col 70 Sector,Unknown,457.78125,-383.0625,-781.625
+Col 70 Sector SM-F c13-1,Col 70 Sector,Unknown,218.40625,-21.53125,-1060.125
+Col 70 Sector SM-G c12-3,Col 70 Sector,Unknown,72.09375,-146.65625,-1065.75
+Col 70 Sector SM-O c21-2,Col 70 Sector,Unknown,401.375,-226.5,-670.90625
+Col 70 Sector SM-O c21-5,Col 70 Sector,Unknown,407.71875,-237.34375,-675.84375
+Col 70 Sector SN-Q b35-0,Col 70 Sector,Unknown,541.84375,-309.96875,-834.34375
+Col 70 Sector SN-R b34-0,Col 70 Sector,Unknown,459.96875,-403.5,-860.875
+Col 70 Sector SN-S d4-12,Col 70 Sector,Unknown,965.5625,-316.375,-1220.84375
+Col 70 Sector SP-E b28-0,Col 70 Sector,Unknown,138.03125,-319.625,-1003.90625
+Col 70 Sector SP-T c18-4,Col 70 Sector,Unknown,785.0625,-273.9375,-790.03125
+Col 70 Sector SQ-A b44-0,Col 70 Sector,Unknown,347.53125,-323.5625,-645.09375
+Col 70 Sector SS-E b30-1,Col 70 Sector,Unknown,740.96875,56.0625,-945.96875
+Col 70 Sector SS-E b30-2,Col 70 Sector,Unknown,752.25,61.34375,-963.875
+Col 70 Sector ST-A a50-0,Col 70 Sector,Unknown,792.1875,-41.15625,-1069.875
+Col 70 Sector ST-F b41-0,Col 70 Sector,Unknown,542.65625,-338.4375,-722.21875
+Col 70 Sector SU-U b33-0,Col 70 Sector,Unknown,336.3125,-243.125,-881.625
+Col 70 Sector SV-J b39-0,Col 70 Sector,Unknown,295.65625,-280.75,-762.25
+Col 70 Sector SV-K b38-0,Col 70 Sector,Unknown,225.1875,-359.03125,-783.9375
+Col 70 Sector SW-Q b35-0,Col 70 Sector,Unknown,98.75,-277.125,-837.5625
+Col 70 Sector SZ-O d6-10,Col 70 Sector,Unknown,295.84375,-331,-1027.34375
+Col 70 Sector SZ-O d6-13,Col 70 Sector,Unknown,282.375,-288.75,-1058.96875
+Col 70 Sector SZ-O d6-2,Col 70 Sector,Unknown,266.875,-334.65625,-1047.28125
+Col 70 Sector SZ-O d6-7,Col 70 Sector,Unknown,306.75,-344.09375,-1041.78125
+Col 70 Sector SZ-Y b17-0,Col 70 Sector,Unknown,699.75,-224.15625,-1222.375
+Col 70 Sector TA-C c14-2,Col 70 Sector,Unknown,424.8125,-383.78125,-998.34375
+Col 70 Sector TB-Z b44-0,Col 70 Sector,Unknown,330.1875,-293.125,-632.25
+Col 70 Sector TC-O c21-2,Col 70 Sector,Unknown,279.25,-314.1875,-677.9375
+Col 70 Sector TD-J b38-0,Col 70 Sector,Unknown,398.78125,-541.53125,-781.75
+Col 70 Sector TD-Y c2-2,Col 70 Sector,Unknown,692.75,-171.4375,-1481.375
+Col 70 Sector TD-Z b30-0,Col 70 Sector,Unknown,400.75,-343.9375,-943.65625
+Col 70 Sector TE-K b37-0,Col 70 Sector,Unknown,840.75,-620.625,-800.5625
+Col 70 Sector TE-Q e5-8,Col 70 Sector,Unknown,418.09375,-224.1875,-688.375
+Col 70 Sector TE-U b21-0,Col 70 Sector,Unknown,371.4375,34.375,-1142.40625
+Col 70 Sector TE-Z a50-0,Col 70 Sector,Unknown,775.9375,-32.0625,-1064.03125
+Col 70 Sector TF-L b25-0,Col 70 Sector,Unknown,157.34375,-181.96875,-1059.28125
+Col 70 Sector TF-N d7-14,Col 70 Sector,Unknown,37.0625,-290.34375,-956.25
+Col 70 Sector TF-T c18-0,Col 70 Sector,Unknown,655.6875,-379.84375,-820.90625
+Col 70 Sector TG-A c15-0,Col 70 Sector,Unknown,280.6875,-355.84375,-957.4375
+Col 70 Sector TH-L c8-1,Col 70 Sector,Unknown,822.84375,-626.15625,-1244.71875
+Col 70 Sector TI-A b30-0,Col 70 Sector,Unknown,358.15625,-403.125,-959.25
+Col 70 Sector TI-R b34-0,Col 70 Sector,Unknown,436.375,-422.40625,-864.125
+Col 70 Sector TI-R c5-4,Col 70 Sector,Unknown,596.46875,-503.125,-1382.5
+Col 70 Sector TJ-F b41-0,Col 70 Sector,Unknown,482.65625,-368.71875,-719.96875
+Col 70 Sector TJ-Q d5-4,Col 70 Sector,Unknown,403.65625,-496.375,-1093.03125
+Col 70 Sector TJ-Q d5-7,Col 70 Sector,Unknown,412.46875,-491.0625,-1110.0625
+Col 70 Sector TJ-Q d5-9,Col 70 Sector,Unknown,389.25,-479.96875,-1074.125
+Col 70 Sector TL-A c15-2,Col 70 Sector,Unknown,363.0625,-315.96875,-952.4375
+Col 70 Sector TM-O c21-6,Col 70 Sector,Unknown,427.6875,-249.9375,-701.0625
+Col 70 Sector TM-W b33-2,Col 70 Sector,Unknown,199.03125,-45.46875,-871.625
+Col 70 Sector TN-R b34-0,Col 70 Sector,Unknown,475.6875,-402.84375,-861.75
+Col 70 Sector TO-W c3-2,Col 70 Sector,Unknown,592.6875,-108.65625,-1447.125
+Col 70 Sector TP-E b28-0,Col 70 Sector,Unknown,156.96875,-323.375,-996.15625
+Col 70 Sector TP-X b18-0,Col 70 Sector,Unknown,720.34375,-182.90625,-1203.125
+Col 70 Sector TS-H a46-1,Col 70 Sector,Unknown,181.9375,-36.78125,-1107.75
+Col 70 Sector TS-J b25-0,Col 70 Sector,Unknown,355.28125,-343.0625,-1060.65625
+Col 70 Sector TS-T d3-5,Col 70 Sector,Unknown,851.53125,-546.71875,-1229.125
+Col 70 Sector TU-N b23-0,Col 70 Sector,Unknown,130.125,-271.03125,-1087.59375
+Col 70 Sector TU-T a53-0,Col 70 Sector,Unknown,140.78125,-34.625,-1028.40625
+Col 70 Sector TV-K b38-0,Col 70 Sector,Unknown,237.03125,-358.8125,-771.21875
+Col 70 Sector TW-Y b44-0,Col 70 Sector,Unknown,275.75,-319.1875,-642.8125
+Col 70 Sector TX-H a46-0,Col 70 Sector,Unknown,195.75,-32.3125,-1112.21875
+Col 70 Sector TX-M c22-2,Col 70 Sector,Unknown,366.90625,-200.96875,-629.65625
+Col 70 Sector TX-S b33-0,Col 70 Sector,Unknown,487.4375,-442.6875,-869.0625
+Col 70 Sector TY-H b26-0,Col 70 Sector,Unknown,280.84375,-331.09375,-1039.6875
+Col 70 Sector TY-O b36-0,Col 70 Sector,Unknown,518.53125,-296.96875,-815.5625
+Col 70 Sector TY-P b35-0,Col 70 Sector,Unknown,437.5,-380.28125,-841.40625
+Col 70 Sector TZ-L c9-4,Col 70 Sector,Unknown,693.625,-130.71875,-1188.0625
+Col 70 Sector TZ-O d6-5,Col 70 Sector,Unknown,398,-311.84375,-1006.53125
+Col 70 Sector UA-L b25-0,Col 70 Sector,Unknown,137.5,-199.6875,-1060.03125
+Col 70 Sector UA-L b38-0,Col 70 Sector,Unknown,302.1875,-333.6875,-782.84375
+Col 70 Sector UB-Z b44-0,Col 70 Sector,Unknown,347.21875,-302.65625,-637.03125
+Col 70 Sector UC-K b25-0,Col 70 Sector,Unknown,460.46875,-300.96875,-1060.25
+Col 70 Sector UC-R b22-1,Col 70 Sector,Unknown,539.03125,-121.5,-1115.59375
+Col 70 Sector UC-X c16-1,Col 70 Sector,Unknown,164.96875,-301.625,-904.3125
+Col 70 Sector UD-Q b35-0,Col 70 Sector,Unknown,495.84375,-354.46875,-825.4375
+Col 70 Sector UF-N d7-12,Col 70 Sector,Unknown,95.96875,-322.6875,-958.3125
+Col 70 Sector UF-W b19-0,Col 70 Sector,Unknown,738.75,-140.9375,-1180.03125
+Col 70 Sector UG-A b44-0,Col 70 Sector,Unknown,305.90625,-358.9375,-646.03125
+Col 70 Sector UG-B b30-0,Col 70 Sector,Unknown,61.3125,-291.5,-958.78125
+Col 70 Sector UG-Z b44-0,Col 70 Sector,Unknown,376.65625,-280.4375,-642.1875
+Col 70 Sector UH-G c12-0,Col 70 Sector,Unknown,55,-219.0625,-1101.1875
+Col 70 Sector UH-O c21-3,Col 70 Sector,Unknown,381.84375,-283.0625,-683.125
+Col 70 Sector UH-O c21-4,Col 70 Sector,Unknown,392.90625,-299.40625,-689.65625
+Col 70 Sector UI-I b26-0,Col 70 Sector,Unknown,377.90625,-301.21875,-1042
+Col 70 Sector UI-J b38-0,Col 70 Sector,Unknown,467.4375,-506.46875,-770.125
+Col 70 Sector UJ-F b28-0,Col 70 Sector,Unknown,336.0625,-243.90625,-1001.59375
+Col 70 Sector UL-A c15-1,Col 70 Sector,Unknown,381.53125,-308.84375,-977.65625
+Col 70 Sector UL-B b30-0,Col 70 Sector,Unknown,100,-284.84375,-961.4375
+Col 70 Sector UN-S c4-0,Col 70 Sector,Unknown,546.59375,-601.21875,-1412.875
+Col 70 Sector UP-E b28-0,Col 70 Sector,Unknown,176.5,-321.71875,-1002.90625
+Col 70 Sector UQ-A b44-0,Col 70 Sector,Unknown,389.3125,-319.125,-661.5
+Col 70 Sector UQ-A c15-0,Col 70 Sector,Unknown,490.25,-268.46875,-949.75
+Col 70 Sector UQ-A c15-2,Col 70 Sector,Unknown,472.90625,-304.59375,-975.6875
+Col 70 Sector UR-F b42-0,Col 70 Sector,Unknown,349.1875,-151.59375,-689.25
+Col 70 Sector UR-G b28-0,Col 70 Sector,Unknown,97.15625,-104.03125,-1004.375
+Col 70 Sector UR-H b40-0,Col 70 Sector,Unknown,182.03125,-315.71875,-742.5625
+Col 70 Sector US-J b25-0,Col 70 Sector,Unknown,379.03125,-343.28125,-1061.28125
+Col 70 Sector US-M c22-4,Col 70 Sector,Unknown,332.59375,-232.5625,-647.5
+Col 70 Sector US-S b33-0,Col 70 Sector,Unknown,472.65625,-451.21875,-878.46875
+Col 70 Sector UT-F b41-0,Col 70 Sector,Unknown,586.53125,-336.5625,-719.09375
+Col 70 Sector UT-F c12-0,Col 70 Sector,Unknown,858.90625,-339.875,-1103.90625
+Col 70 Sector UT-P b35-0,Col 70 Sector,Unknown,430.65625,-386.1875,-828.28125
+Col 70 Sector UU-E b28-0,Col 70 Sector,Unknown,229.125,-285.59375,-1001.09375
+Col 70 Sector UW-G b41-0,Col 70 Sector,Unknown,297.4375,-221.09375,-720.0625
+Col 70 Sector UW-H c11-2,Col 70 Sector,Unknown,148.53125,-232.28125,-1139.75
+Col 70 Sector UW-V b34-0,Col 70 Sector,Unknown,379.375,65.21875,-858.90625
+Col 70 Sector UW-Y b44-0,Col 70 Sector,Unknown,308.46875,-314.0625,-626.75
+Col 70 Sector UX-R c5-0,Col 70 Sector,Unknown,860.59375,-352.96875,-1349.90625
+Col 70 Sector UY-H b26-0,Col 70 Sector,Unknown,298.03125,-339.0625,-1025.09375
+Col 70 Sector UY-I b38-0,Col 70 Sector,Unknown,376.875,-551.46875,-770.90625
+Col 70 Sector UY-N b37-1,Col 70 Sector,Unknown,615.21875,-223.40625,-802.90625
+Col 70 Sector UY-O c7-1,Col 70 Sector,Unknown,789.03125,-243.21875,-1300.8125
+Col 70 Sector UY-P b35-0,Col 70 Sector,Unknown,460.125,-374.78125,-827.03125
+Col 70 Sector UY-Y b30-0,Col 70 Sector,Unknown,391.8125,-348.125,-927
+Col 70 Sector UZ-O d6-8,Col 70 Sector,Unknown,446.875,-305.375,-996.5
+Col 70 Sector VA-D b29-0,Col 70 Sector,Unknown,158.78125,-303,-980.8125
+Col 70 Sector VB-Z b44-0,Col 70 Sector,Unknown,358.59375,-299.875,-643.5625
+Col 70 Sector VC-G c12-2,Col 70 Sector,Unknown,32.46875,-225.71875,-1088.5625
+Col 70 Sector VD-R c5-1,Col 70 Sector,Unknown,579.4375,-543.78125,-1382.3125
+Col 70 Sector VD-Y b31-0,Col 70 Sector,Unknown,519.75,-263.96875,-924
+Col 70 Sector VE-O b36-0,Col 70 Sector,Unknown,397.28125,-381.1875,-819.125
+Col 70 Sector VF-M b24-0,Col 70 Sector,Unknown,118.3125,-264.78125,-1083.5
+Col 70 Sector VF-N d7-12,Col 70 Sector,Unknown,240.03125,-307.53125,-939.9375
+Col 70 Sector VF-S c6-3,Col 70 Sector,Unknown,590.6875,65,-1318.625
+Col 70 Sector VG-B b30-0,Col 70 Sector,Unknown,78.28125,-287.0625,-957.8125
+Col 70 Sector VG-I b40-0,Col 70 Sector,Unknown,315.46875,-259.46875,-740.5
+Col 70 Sector VG-Z c15-0,Col 70 Sector,Unknown,499.875,-222.625,-942.53125
+Col 70 Sector VI-R b34-0,Col 70 Sector,Unknown,475.4375,-405.5,-845.59375
+Col 70 Sector VJ-M b38-0,Col 70 Sector,Unknown,611.75,-201.09375,-784.03125
+Col 70 Sector VJ-N b37-0,Col 70 Sector,Unknown,519.1875,-284.625,-803.09375
+Col 70 Sector VK-N d7-19,Col 70 Sector,Unknown,335.875,-247.53125,-922.875
+Col 70 Sector VL-S c18-0,Col 70 Sector,Unknown,417.40625,-544.75,-820.6875
+Col 70 Sector VM-G b41-0,Col 70 Sector,Unknown,240.5625,-260.34375,-720.34375
+Col 70 Sector VM-R b22-1,Col 70 Sector,Unknown,640.90625,-79.25,-1116.71875
+Col 70 Sector VN-J b25-0,Col 70 Sector,Unknown,373.46875,-351.03125,-1058.75
+Col 70 Sector VN-M c22-3,Col 70 Sector,Unknown,257.03125,-278.4375,-662.625
+Col 70 Sector VR-F c13-4,Col 70 Sector,Unknown,449.96875,43.34375,-1052.625
+Col 70 Sector VR-Y b44-0,Col 70 Sector,Unknown,278,-332.1875,-644.9375
+Col 70 Sector VT-E b16-0,Col 70 Sector,Unknown,370.96875,19.375,-1262
+Col 70 Sector VT-P b35-0,Col 70 Sector,Unknown,441.125,-394.9375,-844.4375
+Col 70 Sector VX-R c5-1,Col 70 Sector,Unknown,909.46875,-356,-1363.59375
+Col 70 Sector VY-C b31-1,Col 70 Sector,Unknown,731.6875,57.75,-930.59375
+Col 70 Sector VY-Z d12,Col 70 Sector,Unknown,706.8125,-165.71875,-1506.78125
+Col 70 Sector WA-D b29-0,Col 70 Sector,Unknown,179.75,-301.375,-981.0625
+Col 70 Sector WA-M b24-0,Col 70 Sector,Unknown,108.34375,-274.625,-1073.75
+Col 70 Sector WA-T c18-1,Col 70 Sector,Unknown,697.28125,-407.65625,-809.9375
+Col 70 Sector WA-T c18-2,Col 70 Sector,Unknown,720.25,-419.46875,-819.78125
+Col 70 Sector WA-W b19-0,Col 70 Sector,Unknown,736.34375,-163.3125,-1179.90625
+Col 70 Sector WB-Z b44-1,Col 70 Sector,Unknown,375.25,-302.3125,-642.34375
+Col 70 Sector WC-N b38-1,Col 70 Sector,Unknown,263.625,-124.375,-780.5625
+Col 70 Sector WC-U c3-0,Col 70 Sector,Unknown,717.28125,-637.34375,-1427.09375
+Col 70 Sector WD-Y b31-0,Col 70 Sector,Unknown,540.75,-263.125,-921.25
+Col 70 Sector WE-F b28-0,Col 70 Sector,Unknown,339.25,-251.71875,-988.59375
+Col 70 Sector WE-N b37-0,Col 70 Sector,Unknown,513.65625,-292.53125,-788.625
+Col 70 Sector WF-X b18-0,Col 70 Sector,Unknown,705.96875,-206.15625,-1190.78125
+Col 70 Sector WG-A c15-2,Col 70 Sector,Unknown,382.59375,-379.4375,-979.96875
+Col 70 Sector WH-F b42-1,Col 70 Sector,Unknown,296.53125,-197.3125,-699.90625
+Col 70 Sector WI-R c5-1,Col 70 Sector,Unknown,702.59375,-486.9375,-1363.625
+Col 70 Sector WI-R c5-7,Col 70 Sector,Unknown,707.1875,-490.96875,-1354.65625
+Col 70 Sector WI-V b5-0,Col 70 Sector,Unknown,657.65625,-265.15625,-1466.125
+Col 70 Sector WJ-G b27-0,Col 70 Sector,Unknown,301.0625,-324.5625,-1009.09375
+Col 70 Sector WJ-Y d1-13,Col 70 Sector,Unknown,591.03125,-95.34375,-1433.53125
+Col 70 Sector WK-B b31-1,Col 70 Sector,Unknown,429.03125,-103.5625,-944.59375
+Col 70 Sector WK-F b40-0,Col 70 Sector,Unknown,255.9375,-559.15625,-740.4375
+Col 70 Sector WM-F c13-0,Col 70 Sector,Unknown,380.03125,12.78125,-1051.375
+Col 70 Sector WM-G b41-0,Col 70 Sector,Unknown,267.21875,-251.96875,-711.21875
+Col 70 Sector WN-J b25-0,Col 70 Sector,Unknown,390.1875,-354.5,-1061.15625
+Col 70 Sector WO-P b35-0,Col 70 Sector,Unknown,434.25,-408.15625,-844.59375
+Col 70 Sector WO-Z a36-2,Col 70 Sector,Unknown,367.40625,-33.9375,-1212.625
+Col 70 Sector WO-Z b29-0,Col 70 Sector,Unknown,262.21875,-470.25,-953.90625
+Col 70 Sector WP-S b22-0,Col 70 Sector,Unknown,375.21875,37.65625,-1124.625
+Col 70 Sector WR-G b13-0,Col 70 Sector,Unknown,940.0625,-339.15625,-1322.34375
+Col 70 Sector WR-G b41-0,Col 70 Sector,Unknown,297.09375,-242.53125,-708.65625
+Col 70 Sector WR-Y b44-0,Col 70 Sector,Unknown,307.9375,-331.6875,-634.4375
+Col 70 Sector WS-S c4-8,Col 70 Sector,Unknown,716.625,-548.90625,-1391.4375
+Col 70 Sector WT-P b35-0,Col 70 Sector,Unknown,455.40625,-403.3125,-842.71875
+Col 70 Sector WT-Z b29-0,Col 70 Sector,Unknown,295.0625,-460.1875,-960.78125
+Col 70 Sector WU-V c16-0,Col 70 Sector,Unknown,697.34375,-574.8125,-877.875
+Col 70 Sector WV-R c19-1,Col 70 Sector,Unknown,779.6875,-274.125,-771.53125
+Col 70 Sector WV-V b19-0,Col 70 Sector,Unknown,700.53125,-179.3125,-1179.71875
+Col 70 Sector WW-P c20-3,Col 70 Sector,Unknown,565.59375,-338.75,-723.5
+Col 70 Sector WX-T b32-0,Col 70 Sector,Unknown,470.5,-508.71875,-891
+Col 70 Sector WY-Q c5-3,Col 70 Sector,Unknown,557.9375,-562.84375,-1383.625
+Col 70 Sector WY-R d4-2,Col 70 Sector,Unknown,737.8125,-584.53125,-1154.21875
+Col 70 Sector XA-D b29-0,Col 70 Sector,Unknown,199.28125,-302.5,-980.6875
+Col 70 Sector XA-N d7-2,Col 70 Sector,Unknown,241.3125,-396.34375,-979.25
+Col 70 Sector XA-N d7-4,Col 70 Sector,Unknown,219.78125,-406.90625,-960.34375
+Col 70 Sector XC-F b42-0,Col 70 Sector,Unknown,277.375,-222.125,-702.15625
+Col 70 Sector XC-U c3-4,Col 70 Sector,Unknown,767.71875,-626,-1429.0625
+Col 70 Sector XC-U c3-6,Col 70 Sector,Unknown,742.40625,-626.96875,-1427.625
+Col 70 Sector XG-B b30-0,Col 70 Sector,Unknown,120.90625,-295.03125,-956.6875
+Col 70 Sector XI-Q c6-0,Col 70 Sector,Unknown,898.5,-343.03125,-1339.15625
+Col 70 Sector XI-Q c6-1,Col 70 Sector,Unknown,901.15625,-331.625,-1322.71875
+Col 70 Sector XJ-F c12-9,Col 70 Sector,Unknown,815.1875,-389.90625,-1082.40625
+Col 70 Sector XJ-P b35-0,Col 70 Sector,Unknown,400.0625,-433.125,-836.25
+Col 70 Sector XJ-W c3-2,Col 70 Sector,Unknown,690.90625,-176.21875,-1451.71875
+Col 70 Sector XK-F b40-0,Col 70 Sector,Unknown,278.625,-563.1875,-744.875
+Col 70 Sector XK-M b24-0,Col 70 Sector,Unknown,198.09375,-239.5625,-1084.375
+Col 70 Sector XK-R b33-0,Col 70 Sector,Unknown,766.15625,-588.15625,-868.25
+Col 70 Sector XN-J b25-0,Col 70 Sector,Unknown,399.15625,-350.6875,-1047
+Col 70 Sector XN-J c9-3,Col 70 Sector,Unknown,821.9375,-626.65625,-1219.5
+Col 70 Sector XN-J c9-4,Col 70 Sector,Unknown,815.625,-625.9375,-1202.125
+Col 70 Sector XN-M c22-1,Col 70 Sector,Unknown,344.53125,-271.625,-628.84375
+Col 70 Sector XN-U c18-4,Col 70 Sector,Unknown,352.90625,-99.0625,-803.90625
+Col 70 Sector XN-V c17-0,Col 70 Sector,Unknown,180.3125,-261.375,-863.21875
+Col 70 Sector XO-P b35-0,Col 70 Sector,Unknown,435.53125,-415.3125,-843.84375
+Col 70 Sector XO-Q d5-7,Col 70 Sector,Unknown,832.75,-370.65625,-1072.71875
+Col 70 Sector XP-D b29-0,Col 70 Sector,Unknown,331,-239.9375,-983.9375
+Col 70 Sector XP-Z b29-0,Col 70 Sector,Unknown,797.46875,-483.78125,-961.125
+Col 70 Sector XQ-K b38-0,Col 70 Sector,Unknown,280.5,-380.625,-781.0625
+Col 70 Sector XQ-M b24-0,Col 70 Sector,Unknown,756.6875,-223.75,-1080.15625
+Col 70 Sector XR-G b13-0,Col 70 Sector,Unknown,959.84375,-341.8125,-1322.40625
+Col 70 Sector XR-Y b44-0,Col 70 Sector,Unknown,318.71875,-343,-644.5
+Col 70 Sector XS-D b43-1,Col 70 Sector,Unknown,280.9375,-184.1875,-679.59375
+Col 70 Sector XS-E b42-0,Col 70 Sector,Unknown,211.03125,-246.21875,-699.78125
+Col 70 Sector XS-E c13-2,Col 70 Sector,Unknown,117.59375,-180.96875,-1063.625
+Col 70 Sector XT-H c10-10,Col 70 Sector,Unknown,692.3125,-625.65625,-1172.75
+Col 70 Sector XT-P b35-0,Col 70 Sector,Unknown,485.6875,-399.3125,-837.875
+Col 70 Sector XT-Z b29-0,Col 70 Sector,Unknown,317.6875,-455.40625,-963.40625
+Col 70 Sector XT-Z d7,Col 70 Sector,Unknown,714.71875,-193.53125,-1509.5625
+Col 70 Sector XV-L d8-18,Col 70 Sector,Unknown,365.03125,-136.96875,-874.4375
+Col 70 Sector XW-Z b43-0,Col 70 Sector,Unknown,282.875,-387.96875,-658.1875
+Col 70 Sector XZ-X b30-0,Col 70 Sector,Unknown,237.21875,-464.78125,-941.4375
+Col 70 Sector YA-M b24-0,Col 70 Sector,Unknown,139.84375,-282.46875,-1082.15625
+Col 70 Sector YA-U b20-0,Col 70 Sector,Unknown,138.375,-180.625,-1159.09375
+Col 70 Sector YC-U c3-6,Col 70 Sector,Unknown,787.0625,-625.4375,-1429.90625
+Col 70 Sector YD-Q c6-0,Col 70 Sector,Unknown,860.875,-383.65625,-1343.59375
+Col 70 Sector YD-R c5-0,Col 70 Sector,Unknown,713.625,-543.6875,-1373.1875
+Col 70 Sector YD-R c5-1,Col 70 Sector,Unknown,720.90625,-512.6875,-1362.5625
+Col 70 Sector YD-R c5-3,Col 70 Sector,Unknown,715.8125,-527.6875,-1373.15625
+Col 70 Sector YE-E b42-0,Col 70 Sector,Unknown,627.59375,-320.875,-698.5625
+Col 70 Sector YE-O b36-0,Col 70 Sector,Unknown,459.96875,-381.84375,-823.34375
+Col 70 Sector YG-B b30-0,Col 70 Sector,Unknown,141.71875,-297.5,-963.875
+Col 70 Sector YG-L a44-0,Col 70 Sector,Unknown,310.5,-37.5,-1125.71875
+Col 70 Sector YG-Q c20-1,Col 70 Sector,Unknown,799.59375,-263.96875,-743.6875
+Col 70 Sector YH-G b41-1,Col 70 Sector,Unknown,257.25,-271,-718.71875
+Col 70 Sector YI-I b26-0,Col 70 Sector,Unknown,457.53125,-304.8125,-1042.875
+Col 70 Sector YI-J c9-0,Col 70 Sector,Unknown,778.375,-699.46875,-1224.34375
+Col 70 Sector YI-M c22-0,Col 70 Sector,Unknown,319.125,-330.34375,-646.8125
+Col 70 Sector YI-M c22-1,Col 70 Sector,Unknown,320.375,-328.15625,-641.21875
+Col 70 Sector YI-M c22-3,Col 70 Sector,Unknown,300.59375,-342.5625,-658.4375
+Col 70 Sector YI-M c22-4,Col 70 Sector,Unknown,299.34375,-314.6875,-631.4375
+Col 70 Sector YI-O a56-0,Col 70 Sector,Unknown,306,-39,-995.15625
+Col 70 Sector YJ-H b39-0,Col 70 Sector,Unknown,417.5,-526.28125,-755.4375
+Col 70 Sector YK-F b40-0,Col 70 Sector,Unknown,298.3125,-561.1875,-740.1875
+Col 70 Sector YK-M b24-0,Col 70 Sector,Unknown,219.75,-244.53125,-1079.59375
+Col 70 Sector YK-R a68-0,Col 70 Sector,Unknown,307.25,-44.40625,-872.75
+Col 70 Sector YK-S b22-1,Col 70 Sector,Unknown,377.71875,28.625,-1106.34375
+Col 70 Sector YL-L d8-6,Col 70 Sector,Unknown,107.9375,-286.84375,-851.15625
+Col 70 Sector YL-Y a64-1,Col 70 Sector,Unknown,209.625,-44.0625,-912.6875
+Col 70 Sector YM-Y b44-0,Col 70 Sector,Unknown,303.71875,-347.8125,-644.96875
+Col 70 Sector YN-S c4-9,Col 70 Sector,Unknown,697.25,-611.875,-1387.1875
+Col 70 Sector YO-F b41-0,Col 70 Sector,Unknown,626.59375,-345.46875,-716.4375
+Col 70 Sector YO-P b35-0,Col 70 Sector,Unknown,457.5625,-423.8125,-844.96875
+Col 70 Sector YP-L c9-1,Col 70 Sector,Unknown,704.03125,-209.875,-1224.65625
+Col 70 Sector YP-L c9-2,Col 70 Sector,Unknown,712.90625,-193.4375,-1220.09375
+Col 70 Sector YQ-S b34-0,Col 70 Sector,Unknown,297.34375,-283.71875,-862.8125
+Col 70 Sector YR-Q c19-1,Col 70 Sector,Unknown,384.84375,-544.59375,-780.96875
+Col 70 Sector YR-Y b44-0,Col 70 Sector,Unknown,337.25,-340.1875,-640
+Col 70 Sector YS-E b42-0,Col 70 Sector,Unknown,221.71875,-254.59375,-689.75
+Col 70 Sector YW-Y b44-0,Col 70 Sector,Unknown,382.34375,-323,-632.90625
+Col 70 Sector YX-D b43-1,Col 70 Sector,Unknown,338.65625,-149.53125,-665.21875
+Col 70 Sector YZ-N b36-0,Col 70 Sector,Unknown,426.28125,-390.375,-814.78125
+Col 70 Sector YZ-W b31-0,Col 70 Sector,Unknown,341.0625,-384.46875,-917.1875
+Col 70 Sector ZA-D b29-0,Col 70 Sector,Unknown,239.4375,-304.5625,-984.125
+Col 70 Sector ZA-M b24-0,Col 70 Sector,Unknown,155.40625,-283.625,-1083
+Col 70 Sector ZA-N d7-11,Col 70 Sector,Unknown,403.03125,-359.15625,-953.5
+Col 70 Sector ZA-N d7-4,Col 70 Sector,Unknown,336.9375,-376.5,-980.96875
+Col 70 Sector ZA-U b20-0,Col 70 Sector,Unknown,172.40625,-168.90625,-1155.96875
+Col 70 Sector ZA-Y b30-0,Col 70 Sector,Unknown,797.875,-461.9375,-943.375
+Col 70 Sector ZC-X b45-0,Col 70 Sector,Unknown,316.625,-322.03125,-619.46875
+Col 70 Sector ZC-X c16-2,Col 70 Sector,Unknown,339.0625,-304.5,-899.34375
+Col 70 Sector ZD-M c22-2,Col 70 Sector,Unknown,276.03125,-353.03125,-656.75
+Col 70 Sector ZD-S b33-0,Col 70 Sector,Unknown,444.65625,-515.8125,-874.21875
+Col 70 Sector ZE-G b27-0,Col 70 Sector,Unknown,319.625,-326.65625,-1010.3125
+Col 70 Sector ZE-Y d1-8,Col 70 Sector,Unknown,699.625,-183,-1427.90625
+Col 70 Sector ZF-K c10-0,Col 70 Sector,Unknown,738.90625,-143.875,-1182.375
+Col 70 Sector ZF-K c10-5,Col 70 Sector,Unknown,757.71875,-139.6875,-1158.875
+Col 70 Sector ZF-N d7-1,Col 70 Sector,Unknown,535.40625,-271.09375,-916.40625
+Col 70 Sector ZH-H b27-0,Col 70 Sector,Unknown,35,-224.75,-1022.78125
+Col 70 Sector ZH-X b45-0,Col 70 Sector,Unknown,359.3125,-303.03125,-621.875
+Col 70 Sector ZH-Y b44-0,Col 70 Sector,Unknown,277.8125,-380.5,-643.3125
+Col 70 Sector ZI-R c5-7,Col 70 Sector,Unknown,818.15625,-499.21875,-1383.875
+Col 70 Sector ZJ-S b7-0,Col 70 Sector,Unknown,675.75,-219.15625,-1444.125
+Col 70 Sector ZK-A a64-4,Col 70 Sector,Unknown,284.09375,-32.65625,-917.5
+Col 70 Sector ZL-Q c7-3,Col 70 Sector,Unknown,582.59375,71.03125,-1287.84375
+Col 70 Sector ZM-I b26-0,Col 70 Sector,Unknown,10.09375,-276.75,-1041.78125
+Col 70 Sector ZM-L b37-0,Col 70 Sector,Unknown,720.375,-481.46875,-801.5
+Col 70 Sector ZM-Q c19-0,Col 70 Sector,Unknown,340.625,-583.53125,-781.9375
+Col 70 Sector ZM-Q c19-1,Col 70 Sector,Unknown,364.3125,-570.5625,-773.125
+Col 70 Sector ZM-Y b44-0,Col 70 Sector,Unknown,326.5625,-347.4375,-629.71875
+Col 70 Sector ZO-Z d7,Col 70 Sector,Unknown,720.40625,-327.28125,-1523.8125
+Col 70 Sector ZO-Z d9,Col 70 Sector,Unknown,728.8125,-321.40625,-1503.8125
+Col 70 Sector ZP-E b28-0,Col 70 Sector,Unknown,278.15625,-321.84375,-1001.9375
+Col 70 Sector ZP-N b23-0,Col 70 Sector,Unknown,198.28125,-299.71875,-1098.21875
+Col 70 Sector ZR-O b37-0,Col 70 Sector,Unknown,370.125,-125.53125,-787.84375
+Col 70 Sector ZR-P b36-0,Col 70 Sector,Unknown,278.875,-211.34375,-816.3125
+Col 70 Sector ZR-Q c19-1,Col 70 Sector,Unknown,441.84375,-511.78125,-763.0625
+Col 70 Sector ZS-E b42-1,Col 70 Sector,Unknown,242.1875,-258.125,-701.28125
+Col 70 Sector ZT-T c18-0,Col 70 Sector,Unknown,97.03125,-259.4375,-821.28125
+Col 70 Sector ZV-M d7-2,Col 70 Sector,Unknown,190.15625,-478.875,-925.75
+Col 70 Sector ZV-M d7-7,Col 70 Sector,Unknown,204.90625,-473.375,-925.21875
+Col 70 Sector ZV-M d7-9,Col 70 Sector,Unknown,227.96875,-464,-926.40625
+Col 70 Sector ZW-Z c14-0,Col 70 Sector,Unknown,361.8125,-444.25,-978.15625
+Col 70 Sector ZX-D c14-4,Col 70 Sector,Unknown,446.03125,41.0625,-1023.6875
+Col 70 Sector ZY-Q c5-8,Col 70 Sector,Unknown,676.25,-579.8125,-1378.0625
+Col 70 Sector ZY-Q c5-9,Col 70 Sector,Unknown,693.375,-580.6875,-1375.78125
+Col 70 Sector ZZ-K b26-1,Col 70 Sector,Unknown,520.53125,-24.28125,-1037.1875
+Col 97 Sector AA-Y c1-0,Col 97 Sector,Unknown,936.96875,-23.34375,-2019.1875
+Col 97 Sector AL-X d1-1,Col 97 Sector,Unknown,799.59375,81.125,-1916.59375
+Col 97 Sector AL-X d1-3,Col 97 Sector,Unknown,775.375,68.15625,-1938.59375
+Col 97 Sector AL-X d1-8,Col 97 Sector,Unknown,784.875,69.59375,-1868.375
+Col 97 Sector AN-I b10-0,Col 97 Sector,Unknown,658.96875,-124.625,-1883.09375
+Col 97 Sector AS-T a33-0,Col 97 Sector,Unknown,1027.5,-43.71875,-1742.5
+Col 97 Sector BC-L c8-3,Col 97 Sector,Unknown,687.375,-140.4375,-1725.25
+Col 97 Sector BH-R b19-0,Col 97 Sector,Unknown,1000.3125,-83.71875,-1679.5625
+Col 97 Sector CI-G b12-0,Col 97 Sector,Unknown,815.3125,27.5,-1839.6875
+Col 97 Sector CQ-U b4-0,Col 97 Sector,Unknown,976.3125,40.15625,-2000.75
+Col 97 Sector DQ-U b4-0,Col 97 Sector,Unknown,1000.34375,40.46875,-2003.46875
+Col 97 Sector EC-T c4-1,Col 97 Sector,Unknown,801.9375,80.40625,-1885.90625
+Col 97 Sector EN-Q b19-0,Col 97 Sector,Unknown,908.5625,-148.15625,-1680.6875
+Col 97 Sector EQ-U b4-0,Col 97 Sector,Unknown,1020.75,39.53125,-2003.03125
+Col 97 Sector FM-U c3-0,Col 97 Sector,Unknown,831.84375,9.84375,-1907.3125
+Col 97 Sector FO-F a41-0,Col 97 Sector,Unknown,755.65625,-42.8125,-1662.46875
+Col 97 Sector FT-G b11-0,Col 97 Sector,Unknown,679.4375,-120.28125,-1862.3125
+Col 97 Sector GI-P b20-0,Col 97 Sector,Unknown,994.0625,-90.8125,-1652.4375
+Col 97 Sector GK-B b15-0,Col 97 Sector,Unknown,754.40625,74.25,-1772.25
+Col 97 Sector GM-V d2-2,Col 97 Sector,Unknown,796.25,23.40625,-1788.21875
+Col 97 Sector GS-C a29-0,Col 97 Sector,Unknown,1048.78125,-33.21875,-1794.46875
+Col 97 Sector GW-C b13-0,Col 97 Sector,Unknown,990.1875,-155.375,-1810.78125
+Col 97 Sector HM-V d2-4,Col 97 Sector,Unknown,840.84375,-20.09375,-1864.15625
+Col 97 Sector HY-F b12-0,Col 97 Sector,Unknown,836.5625,-22.5625,-1833.1875
+Col 97 Sector HY-H c10-1,Col 97 Sector,Unknown,753.71875,-60.71875,-1647.84375
+Col 97 Sector IX-R a34-0,Col 97 Sector,Unknown,808.6875,-44.59375,-1734.90625
+Col 97 Sector JM-U b3-0,Col 97 Sector,Unknown,795.46875,-162.59375,-2022.21875
+Col 97 Sector JR-J b10-0,Col 97 Sector,Unknown,1077.65625,5.4375,-1872.59375
+Col 97 Sector JT-N b8-0,Col 97 Sector,Unknown,835.75,57.1875,-1919.46875
+Col 97 Sector KT-H c10-0,Col 97 Sector,Unknown,790.875,-73.9375,-1664.0625
+Col 97 Sector KZ-E b12-0,Col 97 Sector,Unknown,700.09375,-120.90625,-1839.3125
+Col 97 Sector LP-C b14-0,Col 97 Sector,Unknown,810.90625,12.28125,-1802.6875
+Col 97 Sector MJ-N b8-0,Col 97 Sector,Unknown,815.5,19.21875,-1921.125
+Col 97 Sector MZ-L b9-0,Col 97 Sector,Unknown,824.96875,59.96875,-1888.6875
+Col 97 Sector NF-D b13-0,Col 97 Sector,Unknown,689.6875,-113.9375,-1812.125
+Col 97 Sector NI-T d3-11,Col 97 Sector,Unknown,687.375,-126.59375,-1753.09375
+Col 97 Sector NZ-L b9-0,Col 97 Sector,Unknown,838.34375,57.34375,-1904.03125
+Col 97 Sector OJ-N b8-0,Col 97 Sector,Unknown,857.6875,16.5,-1921.3125
+Col 97 Sector OK-C b14-0,Col 97 Sector,Unknown,830.03125,-16.09375,-1804.6875
+Col 97 Sector OL-S b18-0,Col 97 Sector,Unknown,699.15625,-132.15625,-1698.875
+Col 97 Sector OU-M a37-0,Col 97 Sector,Unknown,766.28125,-28.375,-1704.59375
+Col 97 Sector OV-A b15-0,Col 97 Sector,Unknown,794.5625,11,-1771.34375
+Col 97 Sector OX-T c3-2,Col 97 Sector,Unknown,960.21875,-141.09375,-1918.21875
+Col 97 Sector OZ-Y a30-0,Col 97 Sector,Unknown,1033.1875,-43.9375,-1772.3125
+Col 97 Sector PJ-P c6-1,Col 97 Sector,Unknown,815.5,22.21875,-1823.3125
+Col 97 Sector PL-R b19-0,Col 97 Sector,Unknown,798.96875,-62.28125,-1683.25
+Col 97 Sector QA-C b14-0,Col 97 Sector,Unknown,778.4375,-64.34375,-1801.125
+Col 97 Sector QF-K b10-0,Col 97 Sector,Unknown,820.8125,56.8125,-1870.46875
+Col 97 Sector QG-S b18-0,Col 97 Sector,Unknown,707.125,-158.125,-1701.53125
+Col 97 Sector QS-S c4-1,Col 97 Sector,Unknown,1095.28125,3.78125,-1892.59375
+Col 97 Sector SB-Z b15-0,Col 97 Sector,Unknown,778.9375,-3.84375,-1759.9375
+Col 97 Sector SQ-A b15-0,Col 97 Sector,Unknown,823.25,-20.53125,-1779.34375
+Col 97 Sector SZ-U b17-0,Col 97 Sector,Unknown,1018.78125,-62.15625,-1719.25
+Col 97 Sector TV-L b8-0,Col 97 Sector,Unknown,647.59375,-128.78125,-1912.4375
+Col 97 Sector TY-Q c5-2,Col 97 Sector,Unknown,1066.71875,-9.6875,-1847.71875
+Col 97 Sector UP-M a37-0,Col 97 Sector,Unknown,807.96875,-43.4375,-1703.9375
+Col 97 Sector VG-J a39-0,Col 97 Sector,Unknown,756.4375,-32.65625,-1684.53125
+Col 97 Sector VJ-X a31-0,Col 97 Sector,Unknown,815.96875,-34.96875,-1762.53125
+Col 97 Sector VP-V a32-0,Col 97 Sector,Unknown,775.9375,-33.03125,-1746.5625
+Col 97 Sector VR-Z b14-0,Col 97 Sector,Unknown,678.5625,-123.15625,-1783.6875
+Col 97 Sector WG-I b11-0,Col 97 Sector,Unknown,816.25,45.0625,-1857.28125
+Col 97 Sector WH-P b20-0,Col 97 Sector,Unknown,778.4375,-92.15625,-1645.625
+Col 97 Sector WQ-J b10-0,Col 97 Sector,Unknown,827.375,2,-1878.40625
+Col 97 Sector XC-X b16-0,Col 97 Sector,Unknown,769.21875,-18.53125,-1726.96875
+Col 97 Sector XE-P c6-3,Col 97 Sector,Unknown,1058.71875,-19.59375,-1819.1875
+Col 97 Sector XQ-L c8-2,Col 97 Sector,Unknown,764.09375,-12.59375,-1743.0625
+Col 97 Sector YA-T b18-0,Col 97 Sector,Unknown,1025.75,-75.375,-1691.9375
+Col 97 Sector ZU-U b4-0,Col 97 Sector,Unknown,957.8125,55.78125,-2004.125
+Col 97 Sector ZW-P b7-0,Col 97 Sector,Unknown,800.53125,99.21875,-1940.53125
+Col 121 Sector AA-D b1-0,Col 121 Sector,Unknown,1179.15625,-240.5,-1303.65625
+Col 121 Sector AH-T c4-0,Col 121 Sector,Unknown,887.375,-289,-1136.65625
+Col 121 Sector AP-U b5-0,Col 121 Sector,Unknown,1375.46875,-199.75,-1200.65625
+Col 121 Sector BC-C b29-1,Col 121 Sector,Unknown,887.3125,-232.46875,-700.15625
+Col 121 Sector BH-U b32-0,Col 121 Sector,Unknown,921.0625,-306.5625,-624.8125
+Col 121 Sector BK-D b1-0,Col 121 Sector,Unknown,1276.5,-204.8125,-1299.53125
+Col 121 Sector BL-P b21-0,Col 121 Sector,Unknown,835.6875,-324.65625,-862.9375
+Col 121 Sector BV-X d1-23,Col 121 Sector,Unknown,1364.96875,-163.75,-1171.3125
+Col 121 Sector BV-X d1-24,Col 121 Sector,Unknown,1351.4375,-154.625,-1174.9375
+Col 121 Sector BV-Y e4,Col 121 Sector,Unknown,1284.9375,-265.5625,-1112.96875
+Col 121 Sector CD-F b29-0,Col 121 Sector,Unknown,1018.1875,77.375,-701.0625
+Col 121 Sector CL-X d1-10,Col 121 Sector,Unknown,1073.15625,-300.09375,-1191.34375
+Col 121 Sector CQ-P b21-0,Col 121 Sector,Unknown,900.25,-302.15625,-861.03125
+Col 121 Sector CQ-V c3-1,Col 121 Sector,Unknown,1364.4375,-167.78125,-1161.84375
+Col 121 Sector CV-X d1-30,Col 121 Sector,Unknown,1376.09375,-172.46875,-1149.5
+Col 121 Sector CW-V d2-8,Col 121 Sector,Unknown,929.15625,-207.375,-1075.6875
+Col 121 Sector DG-W c2-0,Col 121 Sector,Unknown,1089.8125,-417.78125,-1200.84375
+Col 121 Sector DI-H b27-5,Col 121 Sector,Unknown,927.0625,-48.25,-741.65625
+Col 121 Sector DL-P b21-0,Col 121 Sector,Unknown,879.03125,-313.9375,-850.78125
+Col 121 Sector DL-R b7-0,Col 121 Sector,Unknown,1357,-163.125,-1164.0625
+Col 121 Sector DP-C c14-10,Col 121 Sector,Unknown,853.1875,-94.46875,-722.4375
+Col 121 Sector DQ-V c3-2,Col 121 Sector,Unknown,1385,-166.71875,-1151.46875
+Col 121 Sector DQ-X d1-0,Col 121 Sector,Unknown,1301.25,-208.78125,-1193.0625
+Col 121 Sector DV-P b21-0,Col 121 Sector,Unknown,958.90625,-280,-863.84375
+Col 121 Sector DZ-D c13-1,Col 121 Sector,Unknown,823.4375,-155.84375,-747.375
+Col 121 Sector EG-P b21-0,Col 121 Sector,Unknown,861.28125,-329.21875,-852.375
+Col 121 Sector EV-S b6-0,Col 121 Sector,Unknown,1390.84375,-186.9375,-1179.65625
+Col 121 Sector FB-X d1-8,Col 121 Sector,Unknown,996.5,-470.6875,-1173.46875
+Col 121 Sector FC-Q b7-0,Col 121 Sector,Unknown,1115.71875,-299.375,-1159.78125
+Col 121 Sector FE-F c12-3,Col 121 Sector,Unknown,832.5,-283.3125,-786.0625
+Col 121 Sector FM-N b22-0,Col 121 Sector,Unknown,800.84375,-343.9375,-842.9375
+Col 121 Sector FM-V d2-9,Col 121 Sector,Unknown,855.53125,-367.4375,-1066.5625
+Col 121 Sector FN-A b30-0,Col 121 Sector,Unknown,915.8125,-223.90625,-682.65625
+Col 121 Sector FP-U c17-5,Col 121 Sector,Unknown,916.78125,-279.25,-584.15625
+Col 121 Sector GB-W d2-14,Col 121 Sector,Unknown,1448.875,-143.53125,-1101.9375
+Col 121 Sector GB-W d2-19,Col 121 Sector,Unknown,1420.59375,-161.4375,-1122.5625
+Col 121 Sector GC-Z b2-0,Col 121 Sector,Unknown,1062.34375,-267.09375,-1263.65625
+Col 121 Sector GM-N b22-0,Col 121 Sector,Unknown,819.84375,-336.71875,-844.21875
+Col 121 Sector GU-D c13-0,Col 121 Sector,Unknown,871.75,-205.9375,-748.40625
+Col 121 Sector GU-D c13-2,Col 121 Sector,Unknown,892.34375,-185.03125,-776.25
+Col 121 Sector GU-D c13-3,Col 121 Sector,Unknown,889.15625,-186.6875,-781.5625
+Col 121 Sector GU-D c13-5,Col 121 Sector,Unknown,883.09375,-192.625,-769.59375
+Col 121 Sector GU-D c13-6,Col 121 Sector,Unknown,875.6875,-200.8125,-758.6875
+Col 121 Sector HA-B b15-0,Col 121 Sector,Unknown,842.125,-388.875,-999.1875
+Col 121 Sector HF-C c14-6,Col 121 Sector,Unknown,839.5625,-167.21875,-744.21875
+Col 121 Sector HI-P b7-0,Col 121 Sector,Unknown,996.6875,-383.84375,-1159.59375
+Col 121 Sector HR-J b10-0,Col 121 Sector,Unknown,1207.375,-422.1875,-1093.28125
+Col 121 Sector HR-V d2-11,Col 121 Sector,Unknown,1169.96875,-298.59375,-1136.71875
+Col 121 Sector HY-H b26-1,Col 121 Sector,Unknown,847.8125,-175.84375,-748.8125
+Col 121 Sector IK-U c17-2,Col 121 Sector,Unknown,936.71875,-308.71875,-581.09375
+Col 121 Sector IL-B b2-0,Col 121 Sector,Unknown,1295.15625,-215.53125,-1281.09375
+Col 121 Sector IN-Y b15-0,Col 121 Sector,Unknown,1138.78125,-480.71875,-980.875
+Col 121 Sector IR-V d2-1,Col 121 Sector,Unknown,1256.15625,-272.96875,-1120.5
+Col 121 Sector IW-T c4-1,Col 121 Sector,Unknown,1420.46875,-176.65625,-1140.875
+Col 121 Sector IX-T d3-13,Col 121 Sector,Unknown,913.53125,-338.0625,-1046.96875
+Col 121 Sector JB-R b7-0,Col 121 Sector,Unknown,1398.28125,-185.625,-1158.125
+Col 121 Sector JC-T d4-18,Col 121 Sector,Unknown,1525.78125,73.40625,-947.90625
+Col 121 Sector JE-F b28-1,Col 121 Sector,Unknown,875.90625,-99.65625,-717.28125
+Col 121 Sector JR-P b8-0,Col 121 Sector,Unknown,1400.84375,-164.4375,-1144.15625
+Col 121 Sector JS-T e3-7,Col 121 Sector,Unknown,897.34375,-104.40625,-705.25
+Col 121 Sector JV-A c15-1,Col 121 Sector,Unknown,917.15625,-100.59375,-690
+Col 121 Sector JX-T d3-13,Col 121 Sector,Unknown,988.4375,-319.875,-1049.875
+Col 121 Sector JX-T d3-16,Col 121 Sector,Unknown,1010.65625,-305.71875,-1060
+Col 121 Sector KA-C c14-6,Col 121 Sector,Unknown,855.8125,-201.3125,-737.625
+Col 121 Sector KA-C c14-7,Col 121 Sector,Unknown,856.25,-202.21875,-743.75
+Col 121 Sector KI-O b8-0,Col 121 Sector,Unknown,1139.1875,-304.75,-1142.71875
+Col 121 Sector KJ-G c11-2,Col 121 Sector,Unknown,943,-419.09375,-848.5
+Col 121 Sector KL-Z b15-0,Col 121 Sector,Unknown,860.59375,-381.78125,-979.25
+Col 121 Sector KO-P c6-2,Col 121 Sector,Unknown,894.9375,-340.5,-1053.28125
+Col 121 Sector KT-P b22-3,Col 121 Sector,Unknown,861.3125,-100.125,-829.46875
+Col 121 Sector KX-T d3-4,Col 121 Sector,Unknown,1084.65625,-284.65625,-1062.34375
+Col 121 Sector LM-V d2-2,Col 121 Sector,Unknown,1319.375,-417.71875,-1098.53125
+Col 121 Sector LN-O b8-0,Col 121 Sector,Unknown,1197.96875,-283.8125,-1131.75
+Col 121 Sector LS-T d3-7,Col 121 Sector,Unknown,1045.4375,-389.21875,-1040.71875
+Col 121 Sector LT-I b25-0,Col 121 Sector,Unknown,804.28125,-284.15625,-783.84375
+Col 121 Sector LV-B c14-0,Col 121 Sector,Unknown,820.71875,-260.375,-741.6875
+Col 121 Sector LW-Z b2-0,Col 121 Sector,Unknown,1317.96875,-203.53125,-1264.78125
+Col 121 Sector MD-S d4-4,Col 121 Sector,Unknown,967.78125,-337.71875,-943.875
+Col 121 Sector MG-G b13-0,Col 121 Sector,Unknown,939.84375,-221.71875,-1040.1875
+Col 121 Sector MN-O b8-0,Col 121 Sector,Unknown,1229.625,-284.25,-1131.65625
+Col 121 Sector MO-N b8-0,Col 121 Sector,Unknown,1016.8125,-381.65625,-1139.875
+Col 121 Sector MV-B c14-4,Col 121 Sector,Unknown,861.75,-229.5625,-728.3125
+Col 121 Sector ND-R c5-1,Col 121 Sector,Unknown,1062.5625,-383.28125,-1104.6875
+Col 121 Sector NN-T d3-4,Col 121 Sector,Unknown,987.90625,-466.71875,-1024.3125
+Col 121 Sector NN-T d3-5,Col 121 Sector,Unknown,993.5,-474.78125,-1032.40625
+Col 121 Sector NT-P c6-1,Col 121 Sector,Unknown,1059.40625,-300.90625,-1063.53125
+Col 121 Sector NY-R d4-11,Col 121 Sector,Unknown,887.0625,-374.65625,-982.75
+Col 121 Sector OF-D c13-1,Col 121 Sector,Unknown,948.0625,-344.5625,-782.5625
+Col 121 Sector PD-K b24-0,Col 121 Sector,Unknown,877.46875,-313.21875,-798.03125
+Col 121 Sector PT-R e4-6,Col 121 Sector,Unknown,935.75,-281.4375,-562.53125
+Col 121 Sector PW-X b16-0,Col 121 Sector,Unknown,919.6875,-351.78125,-958.8125
+Col 121 Sector PX-N b9-0,Col 121 Sector,Unknown,1438.90625,-160.53125,-1123.46875
+Col 121 Sector QJ-Q d5-21,Col 121 Sector,Unknown,945.96875,-293.34375,-865.75
+Col 121 Sector QO-I b25-0,Col 121 Sector,Unknown,856.03125,-300.96875,-783.15625
+Col 121 Sector RD-W b4-0,Col 121 Sector,Unknown,1240.96875,-224.625,-1220.3125
+Col 121 Sector RN-S d4-26,Col 121 Sector,Unknown,1678.90625,-166.34375,-966.375
+Col 121 Sector RT-V b4-0,Col 121 Sector,Unknown,1159.8125,-259.5,-1222.65625
+Col 121 Sector RT-Y c1-1,Col 121 Sector,Unknown,1061.21875,-264.1875,-1262.4375
+Col 121 Sector RU-L b9-0,Col 121 Sector,Unknown,1036.21875,-383.3125,-1121.53125
+Col 121 Sector RU-N b23-2,Col 121 Sector,Unknown,887.34375,-106.375,-822.625
+Col 121 Sector SC-F b14-0,Col 121 Sector,Unknown,1618.09375,-160,-1024.625
+Col 121 Sector SK-N d7-14,Col 121 Sector,Unknown,921.84375,-96.21875,-690.625
+Col 121 Sector SP-O d6-24,Col 121 Sector,Unknown,808,-285.3125,-799.125
+Col 121 Sector SP-T b5-0,Col 121 Sector,Unknown,1019.25,-303.4375,-1204.9375
+Col 121 Sector SX-X b3-0,Col 121 Sector,Unknown,1339.3125,-208.96875,-1243.5
+Col 121 Sector SY-V b4-0,Col 121 Sector,Unknown,1217.125,-244.0625,-1224.1875
+Col 121 Sector TF-N d7-41,Col 121 Sector,Unknown,848.625,-180.65625,-742.21875
+Col 121 Sector TF-N d7-43,Col 121 Sector,Unknown,842.40625,-165.375,-744.96875
+Col 121 Sector TI-M b10-0,Col 121 Sector,Unknown,1477.1875,-143.21875,-1090.0625
+Col 121 Sector TR-G b12-0,Col 121 Sector,Unknown,879.53125,-362.5625,-1062.25
+Col 121 Sector TU-K b10-0,Col 121 Sector,Unknown,1164.4375,-287.6875,-1099
+Col 121 Sector UF-N d7-50,Col 121 Sector,Unknown,908.40625,-112.78125,-694.46875
+Col 121 Sector UQ-B b30-0,Col 121 Sector,Unknown,936.625,-100.09375,-683.09375
+Col 121 Sector UT-A e2,Col 121 Sector,Unknown,1207.25,-45.0625,-1243.9375
+Col 121 Sector UW-G b12-0,Col 121 Sector,Unknown,950.40625,-340.90625,-1060.9375
+Col 121 Sector VA-N d7-12,Col 121 Sector,Unknown,863.625,-195.53125,-743.3125
+Col 121 Sector VA-N d7-2,Col 121 Sector,Unknown,881,-206.59375,-744.125
+Col 121 Sector VA-N d7-38,Col 121 Sector,Unknown,849,-241.8125,-735.3125
+Col 121 Sector VA-N d7-39,Col 121 Sector,Unknown,833.78125,-247.65625,-737.5
+Col 121 Sector VG-H b12-0,Col 121 Sector,Unknown,1040.90625,-300.9375,-1063.40625
+Col 121 Sector VH-W b17-0,Col 121 Sector,Unknown,995.8125,-342.75,-939.8125
+Col 121 Sector VI-W b4-0,Col 121 Sector,Unknown,1359.3125,-203.3125,-1220.84375
+Col 121 Sector VK-T b5-0,Col 121 Sector,Unknown,1046.5625,-307.0625,-1192.6875
+Col 121 Sector WA-N d7-23,Col 121 Sector,Unknown,907.21875,-233.75,-691.96875
+Col 121 Sector WH-F b13-0,Col 121 Sector,Unknown,939.53125,-323.03125,-1039.875
+Col 121 Sector WH-W b17-0,Col 121 Sector,Unknown,1020.34375,-340.625,-943.625
+Col 121 Sector WJ-Z d6,Col 121 Sector,Unknown,1099.25,-240.09375,-1253.53125
+Col 121 Sector WJ-Z d7,Col 121 Sector,Unknown,1085.5,-243.09375,-1251.15625
+Col 121 Sector WJ-Z d9,Col 121 Sector,Unknown,1109.4375,-244.4375,-1238.96875
+Col 121 Sector WL-H b12-0,Col 121 Sector,Unknown,1105.28125,-267.09375,-1058.8125
+Col 121 Sector WP-T b5-0,Col 121 Sector,Unknown,1098,-302.5625,-1186.21875
+Col 121 Sector XC-Y c15-4,Col 121 Sector,Unknown,915.28125,-304.15625,-638.40625
+Col 121 Sector XE-I b25-0,Col 121 Sector,Unknown,919.40625,-343.75,-781.5
+Col 121 Sector XE-X c2-3,Col 121 Sector,Unknown,1253.1875,-219.15625,-1223.15625
+Col 121 Sector XE-Z d8,Col 121 Sector,Unknown,989.5625,-304,-1229.0625
+Col 121 Sector XF-N c7-1,Col 121 Sector,Unknown,975.125,-462.34375,-1021.25
+Col 121 Sector XH-F b13-0,Col 121 Sector,Unknown,959.9375,-321.875,-1041.96875
+Col 121 Sector XP-W c2-1,Col 121 Sector,Unknown,995.5625,-312.03125,-1221.90625
+Col 121 Sector XT-O c7-2,Col 121 Sector,Unknown,1625.15625,-140.375,-1007.1875
+Col 121 Sector XV-D b28-0,Col 121 Sector,Unknown,878.53125,-233.0625,-716.9375
+Col 121 Sector YE-X c2-3,Col 121 Sector,Unknown,1285.8125,-219.65625,-1213
+Col 121 Sector YG-K c9-0,Col 121 Sector,Unknown,939.8125,-342.46875,-943.375
+Col 121 Sector YJ-U b5-0,Col 121 Sector,Unknown,1296.15625,-220.1875,-1201.46875
+Col 121 Sector YL-T c4-2,Col 121 Sector,Unknown,874.75,-242.65625,-1109.4375
+Col 121 Sector ZH-Q b35-3,Col 121 Sector,Unknown,931.125,-178.46875,-551.40625
+Col 121 Sector ZO-A b16-0,Col 121 Sector,Unknown,880.34375,-261.21875,-980.84375
+Col 121 Sector ZR-I c10-1,Col 121 Sector,Unknown,920.21875,-298.59375,-876.5625
+Cone Sector AA-A e2,Cone Sector,Unknown,847.75,81.625,-2032.9375
+Cone Sector AA-A e3,Cone Sector,Unknown,853.4375,75.28125,-2031.5
+Cone Sector BA-Q b5-0,Cone Sector,Unknown,936.3125,40.25,-2020.53125
+Cone Sector BB-W b2-0,Cone Sector,Unknown,831.9375,118.15625,-2083.6875
+Cone Sector BH-L b8-0,Cone Sector,Unknown,819.4375,98.625,-1961.84375
+Cone Sector CC-L b8-0,Cone Sector,Unknown,797.875,79.5625,-1959.75
+Cone Sector CG-O b6-0,Cone Sector,Unknown,880.96875,38.03125,-2001.65625
+Cone Sector CL-X b1-0,Cone Sector,Unknown,850.09375,76.875,-2102.6875
+Cone Sector CM-M b7-0,Cone Sector,Unknown,796.25,39.875,-1980.90625
+Cone Sector CQ-Y c0,Cone Sector,Unknown,845.6875,61.6875,-2071.4375
+Cone Sector CV-Y c0,Cone Sector,Unknown,899.78125,100.15625,-2103.6875
+Cone Sector DL-Y d10,Cone Sector,Unknown,863.75,74.3125,-2058.21875
+Cone Sector DL-Y d11,Cone Sector,Unknown,857.15625,64.03125,-2046.71875
+Cone Sector DL-Y d13,Cone Sector,Unknown,853.96875,85.40625,-2032.1875
+Cone Sector DL-Y d14,Cone Sector,Unknown,857.5625,89.90625,-2032.4375
+Cone Sector DL-Y d15,Cone Sector,Unknown,854.75,84.84375,-2031.71875
+Cone Sector DL-Y d17,Cone Sector,Unknown,847.8125,85.375,-2030.71875
+Cone Sector DL-Y d9,Cone Sector,Unknown,868.46875,89.25,-2057.125
+Cone Sector EL-Y d4,Cone Sector,Unknown,896.25,104.96875,-2079
+Cone Sector EL-Y d5,Cone Sector,Unknown,901.375,87.78125,-2083.21875
+Cone Sector EL-Y d6,Cone Sector,Unknown,933.1875,58,-2029.90625
+Cone Sector EL-Y d7,Cone Sector,Unknown,933.125,63.625,-2032.75
+Cone Sector EM-M b7-0,Cone Sector,Unknown,853.3125,52.40625,-1966.5625
+Cone Sector EQ-Y c0,Cone Sector,Unknown,898.53125,59.15625,-2100.125
+Cone Sector EQ-Y c1,Cone Sector,Unknown,920.78125,62.15625,-2078.625
+Cone Sector FC-L b8-0,Cone Sector,Unknown,855.875,78.03125,-1954.0625
+Cone Sector FL-X b1-0,Cone Sector,Unknown,901.0625,79.71875,-2102.8125
+Cone Sector FL-Y c1,Cone Sector,Unknown,859.875,43.46875,-2073.4375
+Cone Sector FM-M b7-0,Cone Sector,Unknown,862.09375,44.65625,-1973.875
+Cone Sector FN-J b9-0,Cone Sector,Unknown,818.25,99.59375,-1944.375
+Cone Sector GR-W d1-2,Cone Sector,Unknown,802.875,71.6875,-1985.53125
+Cone Sector GW-W c1-1,Cone Sector,Unknown,845.96875,71.125,-2058.53125
+Cone Sector GW-W c1-5,Cone Sector,Unknown,854.71875,83.875,-2025.75
+Cone Sector GW-W c1-6,Cone Sector,Unknown,850.5625,83.09375,-2029.21875
+Cone Sector HR-W d1-14,Cone Sector,Unknown,846.28125,83.53125,-2021.25
+Cone Sector HR-W d1-15,Cone Sector,Unknown,857.84375,79.65625,-2023.8125
+Cone Sector HR-W d1-16,Cone Sector,Unknown,850.625,87.15625,-2020.25
+Cone Sector HR-W d1-17,Cone Sector,Unknown,856.59375,82.8125,-2022.4375
+Cone Sector IR-W d1-0,Cone Sector,Unknown,897.25,63.5625,-2023.5
+Cone Sector IW-W c1-1,Cone Sector,Unknown,913.34375,80.21875,-2056.03125
+Cone Sector IW-W c1-2,Cone Sector,Unknown,896.53125,83.3125,-2042.1875
+Cone Sector JR-V b2-0,Cone Sector,Unknown,896.96875,78.28125,-2074.4375
+Cone Sector JW-V b2-0,Cone Sector,Unknown,939.625,102.28125,-2071.21875
+Cone Sector MC-U b3-0,Cone Sector,Unknown,917.78125,96.90625,-2060.03125
+Cone Sector MN-T c3-0,Cone Sector,Unknown,819.125,95.84375,-1979.1875
+Cone Sector MY-I b9-0,Cone Sector,Unknown,837.5625,35.40625,-1941.40625
+Cone Sector NC-V c2-1,Cone Sector,Unknown,939.96875,59.78125,-2018.25
+Cone Sector NY-I b9-0,Cone Sector,Unknown,857.25,40.09375,-1939.34375
+Cone Sector QS-T b3-0,Cone Sector,Unknown,932.25,68.34375,-2062.78125
+Cone Sector SO-R c4-1,Cone Sector,Unknown,840.4375,65.34375,-1939.71875
+Cone Sector TU-O b6-0,Cone Sector,Unknown,826.8125,99.625,-1991.84375
+Cone Sector TY-R b4-0,Cone Sector,Unknown,900.3125,57.53125,-2032.375
+Cone Sector YE-Q b5-0,Cone Sector,Unknown,920.75,60.125,-2023.5625
+Cone Sector YV-M b7-0,Cone Sector,Unknown,810.125,94.34375,-1967.40625
+Cone Sector ZF-O b6-0,Cone Sector,Unknown,816.71875,52.90625,-1995.75
+Cone Sector ZQ-M b7-0,Cone Sector,Unknown,779.15625,59.28125,-1983.96875
+Horsehead Dark Region AB-W c2-0,Horsehead Dark Sector,Unknown,481.65625,-312.5625,-1298.09375
+Horsehead Dark Region AC-S b5-0,Horsehead Dark Sector,Unknown,478.375,-322.0625,-1283.8125
+Horsehead Dark Region AV-Y d1,Horsehead Dark Sector,Unknown,512.59375,-414.28125,-1366.46875
+Horsehead Dark Region AV-Y d8,Horsehead Dark Sector,Unknown,561.09375,-405.5,-1373.59375
+Horsehead Dark Region BF-O b7-0,Horsehead Dark Sector,Unknown,781.1875,-349.5625,-1228.375
+Horsehead Dark Region BL-X c1-0,Horsehead Dark Sector,Unknown,532.1875,-392.65625,-1314.90625
+Horsehead Dark Region BR-U c3-11,Horsehead Dark Sector,Unknown,523.21875,-251.5,-1232.90625
+Horsehead Dark Region CL-X c1-6,Horsehead Dark Sector,Unknown,549.84375,-408.34375,-1314.375
+Horsehead Dark Region CL-X d1-23,Horsehead Dark Sector,Unknown,730.875,-264.625,-1253.125
+Horsehead Dark Region CM-U c3-2,Horsehead Dark Sector,Unknown,459.59375,-297.71875,-1242.46875
+Horsehead Dark Region CN-Q b6-0,Horsehead Dark Sector,Unknown,477.5,-298.125,-1263.1875
+Horsehead Dark Region CW-V c2-0,Horsehead Dark Sector,Unknown,455.09375,-370.84375,-1267.28125
+Horsehead Dark Region CX-R b5-0,Horsehead Dark Sector,Unknown,484.75,-327.03125,-1271.375
+Horsehead Dark Region DL-X c1-4,Horsehead Dark Sector,Unknown,578.03125,-416.8125,-1336.09375
+Horsehead Dark Region DM-U c3-0,Horsehead Dark Sector,Unknown,497.75,-304.59375,-1262.90625
+Horsehead Dark Region DQ-Y d12,Horsehead Dark Sector,Unknown,596.65625,-481.6875,-1367.34375
+Horsehead Dark Region DQ-Y d2,Horsehead Dark Sector,Unknown,602.90625,-473.65625,-1355.0625
+Horsehead Dark Region DR-V c2-11,Horsehead Dark Sector,Unknown,453.5625,-400.625,-1278.78125
+Horsehead Dark Region DR-V c2-9,Horsehead Dark Sector,Unknown,451.1875,-406.15625,-1300.3125
+Horsehead Dark Region DR-V d2-11,Horsehead Dark Sector,Unknown,483.0625,-255.75,-1184.78125
+Horsehead Dark Region DR-V d2-3,Horsehead Dark Sector,Unknown,493.40625,-264,-1180.71875
+Horsehead Dark Region DW-V c2-0,Horsehead Dark Sector,Unknown,500.4375,-379.4375,-1301.40625
+Horsehead Dark Region EM-U c3-8,Horsehead Dark Sector,Unknown,538.15625,-273.1875,-1247.25
+Horsehead Dark Region EM-U c3-9,Horsehead Dark Sector,Unknown,539.9375,-280.875,-1249.03125
+Horsehead Dark Region EW-K b9-0,Horsehead Dark Sector,Unknown,720.03125,-343.3125,-1201.03125
+Horsehead Dark Region FB-X d1-1,Horsehead Dark Sector,Unknown,642.28125,-378.375,-1280.96875
+Horsehead Dark Region FB-X d1-10,Horsehead Dark Sector,Unknown,610.875,-423.46875,-1294.40625
+Horsehead Dark Region FB-X d1-12,Horsehead Dark Sector,Unknown,645.78125,-378.3125,-1253.625
+Horsehead Dark Region FB-X d1-13,Horsehead Dark Sector,Unknown,642.65625,-371.5,-1265.625
+Horsehead Dark Region FB-X d1-15,Horsehead Dark Sector,Unknown,652.28125,-400.59375,-1298.0625
+Horsehead Dark Region FB-X d1-16,Horsehead Dark Sector,Unknown,647.375,-405.59375,-1294.4375
+Horsehead Dark Region FB-X d1-17,Horsehead Dark Sector,Unknown,635.8125,-398.46875,-1301.09375
+Horsehead Dark Region FB-X d1-18,Horsehead Dark Sector,Unknown,649.1875,-412.03125,-1290.75
+Horsehead Dark Region FB-X d1-2,Horsehead Dark Sector,Unknown,652.28125,-382.46875,-1284.5625
+Horsehead Dark Region FB-X d1-7,Horsehead Dark Sector,Unknown,640.09375,-355.8125,-1244
+Horsehead Dark Region FD-P b7-0,Horsehead Dark Sector,Unknown,535.84375,-254.34375,-1242.1875
+Horsehead Dark Region FG-X c1-2,Horsehead Dark Sector,Unknown,594.96875,-446.5,-1338
+Horsehead Dark Region FH-U c3-0,Horsehead Dark Sector,Unknown,506.5625,-334.125,-1239.28125
+Horsehead Dark Region FM-V d2-19,Horsehead Dark Sector,Unknown,457.65625,-335,-1150.84375
+Horsehead Dark Region FM-V d2-8,Horsehead Dark Sector,Unknown,446.6875,-329.5,-1190.46875
+Horsehead Dark Region FO-N b8-0,Horsehead Dark Sector,Unknown,497.1875,-242.28125,-1222.40625
+Horsehead Dark Region FR-V c2-1,Horsehead Dark Sector,Unknown,522.53125,-411.90625,-1284.1875
+Horsehead Dark Region FR-V c2-2,Horsehead Dark Sector,Unknown,519.71875,-401.84375,-1291.59375
+Horsehead Dark Region FW-W d1-16,Horsehead Dark Sector,Unknown,432.75,-488.84375,-1239
+Horsehead Dark Region FX-S c4-4,Horsehead Dark Sector,Unknown,502.78125,-236.40625,-1220.9375
+Horsehead Dark Region FX-S c4-6,Horsehead Dark Sector,Unknown,498.9375,-244.4375,-1223.4375
+Horsehead Dark Region GB-X d1-10,Horsehead Dark Sector,Unknown,662.46875,-405.59375,-1279.625
+Horsehead Dark Region GB-X d1-11,Horsehead Dark Sector,Unknown,659.125,-414.25,-1242.0625
+Horsehead Dark Region GB-X d1-12,Horsehead Dark Sector,Unknown,673.34375,-394.34375,-1235.875
+Horsehead Dark Region GB-X d1-14,Horsehead Dark Sector,Unknown,687.71875,-393.09375,-1246.25
+Horsehead Dark Region GB-X d1-3,Horsehead Dark Sector,Unknown,694.5625,-398.21875,-1273.34375
+Horsehead Dark Region GB-X d1-6,Horsehead Dark Sector,Unknown,655.6875,-393.3125,-1257.4375
+Horsehead Dark Region GC-T b4-0,Horsehead Dark Sector,Unknown,518.46875,-400.53125,-1302.625
+Horsehead Dark Region GC-U c3-7,Horsehead Dark Sector,Unknown,466.4375,-381.28125,-1246.4375
+Horsehead Dark Region GC-U c3-8,Horsehead Dark Sector,Unknown,457.25,-376.6875,-1225.90625
+Horsehead Dark Region GM-U c3-4,Horsehead Dark Sector,Unknown,631.3125,-302.90625,-1242.78125
+Horsehead Dark Region GM-U c3-5,Horsehead Dark Sector,Unknown,644.125,-274.5,-1233.15625
+Horsehead Dark Region GM-U c3-8,Horsehead Dark Sector,Unknown,632.0625,-303.4375,-1235.90625
+Horsehead Dark Region GO-N b8-0,Horsehead Dark Sector,Unknown,515.625,-239.53125,-1224.90625
+Horsehead Dark Region GR-V c2-5,Horsehead Dark Sector,Unknown,545.5625,-422,-1303.375
+Horsehead Dark Region GR-V d2-20,Horsehead Dark Sector,Unknown,669.53125,-250.78125,-1214.375
+Horsehead Dark Region GR-V d2-23,Horsehead Dark Sector,Unknown,695.8125,-231.71875,-1192.9375
+Horsehead Dark Region HB-O c6-11,Horsehead Dark Sector,Unknown,738.53125,-529.5,-1143.9375
+Horsehead Dark Region HB-X d1-0,Horsehead Dark Sector,Unknown,749.34375,-351.375,-1249.9375
+Horsehead Dark Region HB-X d1-1,Horsehead Dark Sector,Unknown,743.34375,-357.1875,-1245.5
+Horsehead Dark Region HG-X c1-5,Horsehead Dark Sector,Unknown,676.46875,-456.15625,-1309.40625
+Horsehead Dark Region HH-V d2-2,Horsehead Dark Sector,Unknown,435.65625,-347.21875,-1169.21875
+Horsehead Dark Region HJ-N b8-0,Horsehead Dark Sector,Unknown,495.34375,-263.46875,-1221.78125
+Horsehead Dark Region HM-V c2-3,Horsehead Dark Sector,Unknown,502.8125,-453.375,-1278.03125
+Horsehead Dark Region HR-V c2-3,Horsehead Dark Sector,Unknown,579.78125,-421.8125,-1265.15625
+Horsehead Dark Region HW-W d1-12,Horsehead Dark Sector,Unknown,648.09375,-430.9375,-1299.1875
+Horsehead Dark Region HX-T c3-3,Horsehead Dark Sector,Unknown,446.84375,-398.03125,-1241.75
+Horsehead Dark Region HX-T c3-7,Horsehead Dark Sector,Unknown,445.1875,-392.34375,-1231.53125
+Horsehead Dark Region HX-T c3-8,Horsehead Dark Sector,Unknown,445.34375,-390.28125,-1237.5
+Horsehead Dark Region IH-U c3-8,Horsehead Dark Sector,Unknown,638.25,-339.9375,-1239.71875
+Horsehead Dark Region IM-U c3-6,Horsehead Dark Sector,Unknown,721.40625,-287,-1236.28125
+Horsehead Dark Region IM-V c2-2,Horsehead Dark Sector,Unknown,538.78125,-446.5,-1299.3125
+Horsehead Dark Region IM-V c2-7,Horsehead Dark Sector,Unknown,566.40625,-448.21875,-1281.65625
+Horsehead Dark Region IM-V d2-14,Horsehead Dark Sector,Unknown,704.40625,-316.71875,-1196.21875
+Horsehead Dark Region IN-S c4-10,Horsehead Dark Sector,Unknown,493.1875,-330.84375,-1185.0625
+Horsehead Dark Region IO-O b7-0,Horsehead Dark Sector,Unknown,491.96875,-318.375,-1237.96875
+Horsehead Dark Region IR-V c2-0,Horsehead Dark Sector,Unknown,654.78125,-423.6875,-1287.0625
+Horsehead Dark Region IR-V c2-1,Horsehead Dark Sector,Unknown,642.1875,-395.46875,-1284.15625
+Horsehead Dark Region IR-V c2-10,Horsehead Dark Sector,Unknown,644.5625,-424.5625,-1285.75
+Horsehead Dark Region IR-V c2-11,Horsehead Dark Sector,Unknown,646.90625,-422.09375,-1285.75
+Horsehead Dark Region IR-V c2-4,Horsehead Dark Sector,Unknown,630.6875,-420.5625,-1268.9375
+Horsehead Dark Region IR-V c2-5,Horsehead Dark Sector,Unknown,631,-418.8125,-1270.71875
+Horsehead Dark Region IR-V c2-8,Horsehead Dark Sector,Unknown,650.75,-387.25,-1268.0625
+Horsehead Dark Region IR-V c2-9,Horsehead Dark Sector,Unknown,649.625,-423.53125,-1281.1875
+Horsehead Dark Region IW-V c2-7,Horsehead Dark Sector,Unknown,712.625,-384.5625,-1273.15625
+Horsehead Dark Region IW-V c2-8,Horsehead Dark Sector,Unknown,716.28125,-382.75,-1275.1875
+Horsehead Dark Region IW-W d1-11,Horsehead Dark Sector,Unknown,696.25,-445.5625,-1263.96875
+Horsehead Dark Region IW-W d1-3,Horsehead Dark Sector,Unknown,726.875,-435.5625,-1252.59375
+Horsehead Dark Region IW-W d1-6,Horsehead Dark Sector,Unknown,687.21875,-470.84375,-1292.9375
+Horsehead Dark Region IX-T c3-2,Horsehead Dark Sector,Unknown,457.1875,-396.28125,-1257.875
+Horsehead Dark Region IX-T c3-4,Horsehead Dark Sector,Unknown,463.4375,-395.28125,-1262.71875
+Horsehead Dark Region JB-X c1-1,Horsehead Dark Sector,Unknown,667.59375,-467.84375,-1315.9375
+Horsehead Dark Region JB-X c1-3,Horsehead Dark Sector,Unknown,666.78125,-474.96875,-1325.1875
+Horsehead Dark Region JH-J b10-0,Horsehead Dark Sector,Unknown,776.09375,-313.65625,-1176.125
+Horsehead Dark Region JM-V c2-5,Horsehead Dark Sector,Unknown,614.375,-433.15625,-1284.78125
+Horsehead Dark Region JM-V c2-6,Horsehead Dark Sector,Unknown,582.34375,-425.9375,-1294.65625
+Horsehead Dark Region JR-V c2-7,Horsehead Dark Sector,Unknown,681,-420.96875,-1294.25
+Horsehead Dark Region JR-V c2-8,Horsehead Dark Sector,Unknown,675.46875,-421.03125,-1291
+Horsehead Dark Region JR-V c2-9,Horsehead Dark Sector,Unknown,676.125,-418.09375,-1296.6875
+Horsehead Dark Region JR-W d1-1,Horsehead Dark Sector,Unknown,607.9375,-573.09375,-1229.0625
+Horsehead Dark Region JR-W d1-7,Horsehead Dark Sector,Unknown,644.3125,-507.96875,-1304.9375
+Horsehead Dark Region JR-W d1-9,Horsehead Dark Sector,Unknown,642.65625,-516.4375,-1293.71875
+Horsehead Dark Region JW-V c2-4,Horsehead Dark Sector,Unknown,766.71875,-373.5625,-1281.90625
+Horsehead Dark Region JW-W d1-4,Horsehead Dark Sector,Unknown,757.71875,-428.21875,-1244.59375
+Horsehead Dark Region JX-S c4-0,Horsehead Dark Sector,Unknown,655.375,-260.21875,-1220.53125
+Horsehead Dark Region JX-S c4-1,Horsehead Dark Sector,Unknown,685.90625,-235.46875,-1223.5
+Horsehead Dark Region KC-U c3-9,Horsehead Dark Sector,Unknown,639.78125,-366.8125,-1237.90625
+Horsehead Dark Region KH-U c3-11,Horsehead Dark Sector,Unknown,701.4375,-310,-1237.28125
+Horsehead Dark Region KH-V c2-8,Horsehead Dark Sector,Unknown,552.875,-465.0625,-1296.5
+Horsehead Dark Region KI-S c4-9,Horsehead Dark Sector,Unknown,456.84375,-356.125,-1222.03125
+Horsehead Dark Region KM-V c2-10,Horsehead Dark Sector,Unknown,647.6875,-453.65625,-1296.78125
+Horsehead Dark Region KM-V c2-4,Horsehead Dark Sector,Unknown,625.28125,-442,-1302.875
+Horsehead Dark Region KM-V c2-9,Horsehead Dark Sector,Unknown,646.34375,-454.0625,-1300.21875
+Horsehead Dark Region KR-W d1-6,Horsehead Dark Sector,Unknown,708.8125,-522.65625,-1296.90625
+Horsehead Dark Region KW-W c1-3,Horsehead Dark Sector,Unknown,636.84375,-524.75,-1309.375
+Horsehead Dark Region KZ-C b14-0,Horsehead Dark Sector,Unknown,639.90625,-242.1875,-1104.4375
+Horsehead Dark Region LD-S c4-11,Horsehead Dark Sector,Unknown,452.03125,-401.125,-1196.625
+Horsehead Dark Region LD-S c4-7,Horsehead Dark Sector,Unknown,449.9375,-387.6875,-1210.125
+Horsehead Dark Region LM-V c2-1,Horsehead Dark Sector,Unknown,662.125,-437.59375,-1267.65625
+Horsehead Dark Region LM-V c2-10,Horsehead Dark Sector,Unknown,668.0625,-446.96875,-1293.71875
+Horsehead Dark Region LM-V c2-2,Horsehead Dark Sector,Unknown,659.5,-442.8125,-1273.28125
+Horsehead Dark Region LM-V c2-3,Horsehead Dark Sector,Unknown,657.0625,-444.96875,-1279.65625
+Horsehead Dark Region LM-V c2-4,Horsehead Dark Sector,Unknown,662.15625,-439.21875,-1276.03125
+Horsehead Dark Region LM-V c2-5,Horsehead Dark Sector,Unknown,687.65625,-446.375,-1283.53125
+Horsehead Dark Region LM-V c2-6,Horsehead Dark Sector,Unknown,681.5625,-447.28125,-1289.40625
+Horsehead Dark Region MC-U c3-2,Horsehead Dark Sector,Unknown,697.1875,-372.3125,-1228.53125
+Horsehead Dark Region MC-U c3-6,Horsehead Dark Sector,Unknown,716.46875,-352.3125,-1245.34375
+Horsehead Dark Region MM-V c2-6,Horsehead Dark Sector,Unknown,715.84375,-446.15625,-1270.625
+Horsehead Dark Region MN-S c4-6,Horsehead Dark Sector,Unknown,638.03125,-324.875,-1224.90625
+Horsehead Dark Region MO-O b7-0,Horsehead Dark Sector,Unknown,560.28125,-322.34375,-1242.6875
+Horsehead Dark Region MO-P b6-0,Horsehead Dark Sector,Unknown,476.71875,-399.0625,-1262.9375
+Horsehead Dark Region MP-M b8-0,Horsehead Dark Sector,Unknown,450.21875,-341.6875,-1207.46875
+Horsehead Dark Region MR-W c1-4,Horsehead Dark Sector,Unknown,636.71875,-555.3125,-1315.84375
+Horsehead Dark Region MS-T c3-0,Horsehead Dark Sector,Unknown,570.5,-430.90625,-1258.09375
+Horsehead Dark Region MS-T c3-10,Horsehead Dark Sector,Unknown,565.90625,-446.28125,-1251.71875
+Horsehead Dark Region MT-Q c5-2,Horsehead Dark Sector,Unknown,478.15625,-326.65625,-1184.25
+Horsehead Dark Region MT-Q c5-3,Horsehead Dark Sector,Unknown,455.8125,-330.1875,-1185
+Horsehead Dark Region MX-T c3-1,Horsehead Dark Sector,Unknown,618.71875,-423.625,-1262.5625
+Horsehead Dark Region NC-U c3-0,Horsehead Dark Sector,Unknown,736.0625,-380.375,-1263.21875
+Horsehead Dark Region NC-U c3-7,Horsehead Dark Sector,Unknown,769.0625,-359.46875,-1237.3125
+Horsehead Dark Region ND-R c5-8,Horsehead Dark Sector,Unknown,671.40625,-228.78125,-1151.1875
+Horsehead Dark Region NH-V c2-3,Horsehead Dark Sector,Unknown,690.09375,-500.21875,-1286.53125
+Horsehead Dark Region NJ-O b7-0,Horsehead Dark Sector,Unknown,546.96875,-330.65625,-1228.71875
+Horsehead Dark Region NO-O b7-0,Horsehead Dark Sector,Unknown,576.65625,-320.8125,-1242.6875
+Horsehead Dark Region NX-T c3-0,Horsehead Dark Sector,Unknown,671.25,-423.9375,-1245.4375
+Horsehead Dark Region NX-T c3-10,Horsehead Dark Sector,Unknown,664.03125,-407.15625,-1255.0625
+Horsehead Dark Region NX-T c3-11,Horsehead Dark Sector,Unknown,658.9375,-405.40625,-1254.34375
+Horsehead Dark Region NX-T c3-8,Horsehead Dark Sector,Unknown,672.75,-415.90625,-1263.8125
+Horsehead Dark Region NX-T c3-9,Horsehead Dark Sector,Unknown,660.875,-415.28125,-1260.46875
+Horsehead Dark Region OC-V c2-6,Horsehead Dark Sector,Unknown,645.96875,-521.5625,-1278.53125
+Horsehead Dark Region OC-V c2-9,Horsehead Dark Sector,Unknown,644,-543.78125,-1302.125
+Horsehead Dark Region OS-T c3-10,Horsehead Dark Sector,Unknown,644.71875,-459.875,-1246.21875
+Horsehead Dark Region OS-T c3-6,Horsehead Dark Sector,Unknown,653.4375,-426.25,-1262.15625
+Horsehead Dark Region OS-T c3-7,Horsehead Dark Sector,Unknown,652.375,-427.09375,-1264.4375
+Horsehead Dark Region OT-O b7-0,Horsehead Dark Sector,Unknown,639.46875,-291.625,-1241.75
+Horsehead Dark Region OX-U c2-0,Horsehead Dark Sector,Unknown,537.375,-555.78125,-1274.28125
+Horsehead Dark Region OX-U c2-4,Horsehead Dark Sector,Unknown,561.34375,-572.25,-1280.5625
+Horsehead Dark Region OX-U d2-11,Horsehead Dark Sector,Unknown,687.75,-558.9375,-1212.03125
+Horsehead Dark Region PJ-N b8-0,Horsehead Dark Sector,Unknown,673.5,-247.125,-1211.28125
+Horsehead Dark Region PN-S c4-0,Horsehead Dark Sector,Unknown,737.09375,-343.6875,-1224.65625
+Horsehead Dark Region PN-T c3-9,Horsehead Dark Sector,Unknown,603.15625,-487.5625,-1246.09375
+Horsehead Dark Region PN-T d3-4,Horsehead Dark Sector,Unknown,786.75,-357.03125,-1133.5
+Horsehead Dark Region PP-M b8-0,Horsehead Dark Sector,Unknown,499.59375,-339.5,-1220.875
+Horsehead Dark Region PS-T c3-1,Horsehead Dark Sector,Unknown,666.34375,-443.625,-1258.78125
+Horsehead Dark Region PS-T c3-2,Horsehead Dark Sector,Unknown,667.53125,-440.65625,-1243.34375
+Horsehead Dark Region PT-R c4-10,Horsehead Dark Sector,Unknown,426.15625,-466.6875,-1192.09375
+Horsehead Dark Region PT-R c4-4,Horsehead Dark Sector,Unknown,431.78125,-479.5,-1211.90625
+Horsehead Dark Region PU-M b8-0,Horsehead Dark Sector,Unknown,538.53125,-322.78125,-1224.5625
+Horsehead Dark Region QJ-P c6-4,Horsehead Dark Sector,Unknown,648.75,-241.25,-1134.28125
+Horsehead Dark Region QO-O b7-0,Horsehead Dark Sector,Unknown,640.28125,-323.75,-1239.5625
+Horsehead Dark Region QS-T c3-3,Horsehead Dark Sector,Unknown,724.3125,-448.625,-1239.75
+Horsehead Dark Region RD-T c3-5,Horsehead Dark Sector,Unknown,525.84375,-561.625,-1248.78125
+Horsehead Dark Region RI-T c3-5,Horsehead Dark Sector,Unknown,604.375,-509.15625,-1248.78125
+Horsehead Dark Region SA-L b9-0,Horsehead Dark Sector,Unknown,534.875,-318.40625,-1203.34375
+Horsehead Dark Region SG-I b11-0,Horsehead Dark Sector,Unknown,526.40625,-242.53125,-1159.09375
+Horsehead Dark Region SP-V b3-0,Horsehead Dark Sector,Unknown,485.09375,-308.375,-1311.8125
+Horsehead Dark Region TV-K b9-0,Horsehead Dark Sector,Unknown,496.53125,-341.71875,-1199.90625
+Horsehead Dark Region TZ-P c5-3,Horsehead Dark Sector,Unknown,425.125,-469.5625,-1167.5
+Horsehead Dark Region VJ-R c4-5,Horsehead Dark Sector,Unknown,531.5625,-559.25,-1217.375
+Horsehead Dark Region VO-Q c5-7,Horsehead Dark Sector,Unknown,746.84375,-365.53125,-1174.09375
+Horsehead Dark Region VP-L b9-0,Horsehead Dark Sector,Unknown,697.3125,-263.21875,-1199.8125
+Horsehead Dark Region WU-M b8-0,Horsehead Dark Sector,Unknown,676.65625,-320.625,-1221.78125
+Horsehead Dark Region XE-Z c5,Horsehead Dark Sector,Unknown,533.53125,-404.9375,-1372.5625
+Horsehead Dark Region XY-P b6-0,Horsehead Dark Sector,Unknown,776.9375,-359.09375,-1262.96875
+Horsehead Dark Region YE-Z c9,Horsehead Dark Sector,Unknown,548.1875,-416.6875,-1365.59375
+Horsehead Dark Region YJ-O b7-0,Horsehead Dark Sector,Unknown,759.3125,-344.375,-1242.96875
+Horsehead Dark Region YL-I b11-0,Horsehead Dark Sector,Unknown,680.375,-223.84375,-1162.21875
+Horsehead Dark Region ZE-Q c5-7,Horsehead Dark Sector,Unknown,748.21875,-448.5,-1174.90625
+Horsehead Dark Region ZG-S b5-0,Horsehead Dark Sector,Unknown,513.5,-298.15625,-1284.15625
+M41 Sector DB-O b22-0,M41 Sector,Unknown,1477.15625,-299.875,-1281.875
+M41 Sector DD-G c12-7,M41 Sector,Unknown,1755.59375,-127.96875,-1250.78125
+M41 Sector FM-U d3-11,M41 Sector,Unknown,1825.75,-121.46875,-1442.6875
+M41 Sector MV-P b8-0,M41 Sector,Unknown,1576.03125,-159.53125,-1579.875
+M41 Sector OT-Z b29-0,M41 Sector,Unknown,1539.09375,-324.6875,-1122.96875
+M41 Sector PZ-O d6-17,M41 Sector,Unknown,1502.34375,-261.6875,-1224.71875
+M41 Sector QF-M c8-1,M41 Sector,Unknown,1456.375,-342.09375,-1422.6875
+M41 Sector QI-W b4-0,M41 Sector,Unknown,1855.09375,-220.28125,-1662.78125
+M41 Sector QO-X c2-0,M41 Sector,Unknown,1725.28125,-182.125,-1652.9375
+M41 Sector UL-G b13-0,M41 Sector,Unknown,1739.65625,-224.21875,-1483.8125
+M41 Sector XJ-Z d5,M41 Sector,Unknown,1779.28125,-303.53125,-1646.875
+M41 Sector XO-P c6-1,M41 Sector,Unknown,2012.0625,-380.875,-1494.78125
+M41 Sector ZT-S b19-0,M41 Sector,Unknown,1519.25,-363.25,-1339.34375
+NGC 1647 Sector KI-R c5-1,NGC 1647 Sector,Unknown,17.90625,-340.40625,-1662.65625
+NGC 1647 Sector KS-T d3-2,NGC 1647 Sector,Unknown,0.75,-388.5,-1591.71875
+NGC 1647 Sector QI-T d3-3,NGC 1647 Sector,Unknown,117.125,-525.09375,-1595.875
+NGC 2264 Sector AA-Q b21-0,NGC 2264 Sector,Unknown,496.96875,39.4375,-2061.9375
+NGC 2264 Sector AG-F b27-0,NGC 2264 Sector,Unknown,497.34375,33.1875,-1942.5
+NGC 2264 Sector AG-H b26-0,NGC 2264 Sector,Unknown,1136.0625,36.21875,-1961.8125
+NGC 2264 Sector AG-W b44-0,NGC 2264 Sector,Unknown,736.0625,-141.9375,-1564.6875
+NGC 2264 Sector AH-L d8-2,NGC 2264 Sector,Unknown,514.59375,39.5625,-1859.625
+NGC 2264 Sector AO-W c16-0,NGC 2264 Sector,Unknown,515.28125,30.65625,-1809.40625
+NGC 2264 Sector AO-W c16-2,NGC 2264 Sector,Unknown,520.8125,39.1875,-1818.96875
+NGC 2264 Sector AV-O d6-18,NGC 2264 Sector,Unknown,1161.1875,41.9375,-1972.03125
+NGC 2264 Sector AZ-B b28-0,NGC 2264 Sector,Unknown,619.40625,-141.6875,-1921
+NGC 2264 Sector BF-B a86-0,NGC 2264 Sector,Unknown,567.40625,-43,-1604.84375
+NGC 2264 Sector BG-W d2-1,NGC 2264 Sector,Unknown,780.78125,169.1875,-2331.96875
+NGC 2264 Sector BK-F c12-0,NGC 2264 Sector,Unknown,1353.21875,38.75,-1999.6875
+NGC 2264 Sector BU-K b24-0,NGC 2264 Sector,Unknown,1317.59375,39.125,-2001.28125
+NGC 2264 Sector BW-V b18-0,NGC 2264 Sector,Unknown,372.8125,114.84375,-2119.53125
+NGC 2264 Sector CE-L b10-0,NGC 2264 Sector,Unknown,467.6875,48.4375,-2298.875
+NGC 2264 Sector CE-L b10-1,NGC 2264 Sector,Unknown,469.21875,48.59375,-2302.375
+NGC 2264 Sector CE-V c17-0,NGC 2264 Sector,Unknown,578.15625,96.59375,-1782.71875
+NGC 2264 Sector CJ-W c16-1,NGC 2264 Sector,Unknown,503.09375,4.28125,-1787.6875
+NGC 2264 Sector CV-O d6-17,NGC 2264 Sector,Unknown,1340.1875,37.375,-2000.6875
+NGC 2264 Sector CW-K c9-0,NGC 2264 Sector,Unknown,905.84375,80.03125,-2135.96875
+NGC 2264 Sector CX-S b33-0,NGC 2264 Sector,Unknown,500.84375,19.15625,-1801.71875
+NGC 2264 Sector DA-S b20-0,NGC 2264 Sector,Unknown,1203.65625,72.9375,-2069.125
+NGC 2264 Sector DJ-F a70-1,NGC 2264 Sector,Unknown,512.1875,-28.59375,-1773.125
+NGC 2264 Sector DO-F b41-0,NGC 2264 Sector,Unknown,555.21875,112.125,-1630.34375
+NGC 2264 Sector DR-D b28-0,NGC 2264 Sector,Unknown,519.71875,38.96875,-1922.0625
+NGC 2264 Sector DS-R c5-1,NGC 2264 Sector,Unknown,477.4375,25.15625,-2265.3125
+NGC 2264 Sector DS-R c5-2,NGC 2264 Sector,Unknown,475.0625,28.65625,-2270.34375
+NGC 2264 Sector EG-E b28-0,NGC 2264 Sector,Unknown,657.1875,100.78125,-1924.46875
+NGC 2264 Sector EG-O b22-0,NGC 2264 Sector,Unknown,500.5625,38.65625,-2043.625
+NGC 2264 Sector EL-A b29-0,NGC 2264 Sector,Unknown,1175.65625,-120.9375,-1900.78125
+NGC 2264 Sector EN-J d9-15,NGC 2264 Sector,Unknown,505,-16,-1778.90625
+NGC 2264 Sector EP-U a75-0,NGC 2264 Sector,Unknown,518.125,-41.1875,-1710.03125
+NGC 2264 Sector FB-R b33-0,NGC 2264 Sector,Unknown,1197.71875,-165.9375,-1787.375
+NGC 2264 Sector FC-U d3-6,NGC 2264 Sector,Unknown,471.5625,29.90625,-2243.75
+NGC 2264 Sector FH-F b27-0,NGC 2264 Sector,Unknown,1119,19.21875,-1943.0625
+NGC 2264 Sector FL-G b39-0,NGC 2264 Sector,Unknown,717.96875,-180.9375,-1683.625
+NGC 2264 Sector FW-T c17-0,NGC 2264 Sector,Unknown,1197.65625,-172.15625,-1766.6875
+NGC 2264 Sector GB-V c16-1,NGC 2264 Sector,Unknown,1140.21875,-271.65625,-1802.75
+NGC 2264 Sector GF-Y b43-0,NGC 2264 Sector,Unknown,984.40625,-112.71875,-1578.1875
+NGC 2264 Sector GG-Q b21-0,NGC 2264 Sector,Unknown,1184.3125,64.9375,-2046.25
+NGC 2264 Sector GI-J d9-7,NGC 2264 Sector,Unknown,518.125,-29.875,-1759.84375
+NGC 2264 Sector GK-J b11-0,NGC 2264 Sector,Unknown,470.09375,36.0625,-2278.96875
+NGC 2264 Sector GP-U c17-0,NGC 2264 Sector,Unknown,511.28125,-21.71875,-1777.09375
+NGC 2264 Sector GP-U c17-1,NGC 2264 Sector,Unknown,510.8125,-17.71875,-1776.625
+NGC 2264 Sector GU-D b42-0,NGC 2264 Sector,Unknown,539.5625,113.84375,-1618.03125
+NGC 2264 Sector GV-Y b16-0,NGC 2264 Sector,Unknown,498.375,38.09375,-2159.125
+NGC 2264 Sector GY-H d10-7,NGC 2264 Sector,Unknown,559.15625,110.8125,-1661
+NGC 2264 Sector HE-N c21-3,NGC 2264 Sector,Unknown,780.59375,-101.40625,-1623.375
+NGC 2264 Sector HG-G b39-0,NGC 2264 Sector,Unknown,726.15625,-203.53125,-1668.34375
+NGC 2264 Sector HJ-G b40-0,NGC 2264 Sector,Unknown,515.5625,-1.875,-1663
+NGC 2264 Sector HK-J b11-0,NGC 2264 Sector,Unknown,475.03125,38.3125,-2284.46875
+NGC 2264 Sector HM-M b23-0,NGC 2264 Sector,Unknown,490.5625,44.21875,-2017.40625
+NGC 2264 Sector HM-V d2-6,NGC 2264 Sector,Unknown,600,-150.15625,-2288.3125
+NGC 2264 Sector HW-Q b33-0,NGC 2264 Sector,Unknown,1199.21875,-203.1875,-1803.65625
+NGC 2264 Sector HW-Z b2-0,NGC 2264 Sector,Unknown,812.15625,96.78125,-2456.03125
+NGC 2264 Sector HX-B b29-0,NGC 2264 Sector,Unknown,518.5625,39.28125,-1899.0625
+NGC 2264 Sector HY-P b35-0,NGC 2264 Sector,Unknown,574.25,92.15625,-1758.6875
+NGC 2264 Sector IF-T c18-1,NGC 2264 Sector,Unknown,578.0625,91.46875,-1727.75
+NGC 2264 Sector IM-M b23-0,NGC 2264 Sector,Unknown,500.25,38.53125,-2023.125
+NGC 2264 Sector IO-H b39-0,NGC 2264 Sector,Unknown,510.46875,-62.5625,-1668.28125
+NGC 2264 Sector IP-D a71-0,NGC 2264 Sector,Unknown,517.46875,-34.9375,-1763.59375
+NGC 2264 Sector IV-S a76-0,NGC 2264 Sector,Unknown,517.8125,-43.0625,-1704.59375
+NGC 2264 Sector IY-H d10-2,NGC 2264 Sector,Unknown,691.09375,98.09375,-1636.625
+NGC 2264 Sector IY-Q b34-0,NGC 2264 Sector,Unknown,500.40625,-4.59375,-1782.25
+NGC 2264 Sector JC-S c18-0,NGC 2264 Sector,Unknown,1179,-182.625,-1742.0625
+NGC 2264 Sector JH-C b29-0,NGC 2264 Sector,Unknown,641.4375,87.3125,-1904.9375
+NGC 2264 Sector JH-X b17-0,NGC 2264 Sector,Unknown,1043.375,69.9375,-2135.84375
+NGC 2264 Sector JM-Q b33-0,NGC 2264 Sector,Unknown,1159.875,-243.09375,-1799.125
+NGC 2264 Sector JN-M b23-0,NGC 2264 Sector,Unknown,1035.375,39.40625,-2020.59375
+NGC 2264 Sector JR-M d7-4,NGC 2264 Sector,Unknown,1269.5,-135.375,-1869.90625
+NGC 2264 Sector JS-M b23-0,NGC 2264 Sector,Unknown,1076.84375,56.5,-2021.5
+NGC 2264 Sector JV-O b22-0,NGC 2264 Sector,Unknown,716.46875,107.75,-2040.34375
+NGC 2264 Sector KB-X b17-0,NGC 2264 Sector,Unknown,497.65625,37.90625,-2140
+NGC 2264 Sector KD-I d10-9,NGC 2264 Sector,Unknown,1037.125,182.0625,-1704.96875
+NGC 2264 Sector KG-N b23-0,NGC 2264 Sector,Unknown,700.65625,119.46875,-2020.53125
+NGC 2264 Sector KH-Q b33-0,NGC 2264 Sector,Unknown,1146,-256.5625,-1789.46875
+NGC 2264 Sector KH-V e2-0,NGC 2264 Sector,Unknown,977.25,108.3125,-2083.46875
+NGC 2264 Sector KH-X b17-0,NGC 2264 Sector,Unknown,1066.71875,73.1875,-2132.9375
+NGC 2264 Sector KI-D b28-0,NGC 2264 Sector,Unknown,1113.71875,14.5,-1913.5625
+NGC 2264 Sector KM-X b17-0,NGC 2264 Sector,Unknown,1095.84375,77.71875,-2139.59375
+NGC 2264 Sector KN-M b23-0,NGC 2264 Sector,Unknown,1059.03125,40.53125,-2020.8125
+NGC 2264 Sector KO-H d10-6,NGC 2264 Sector,Unknown,514.71875,-62.21875,-1679.4375
+NGC 2264 Sector KO-H d10-7,NGC 2264 Sector,Unknown,512.21875,-50.84375,-1690.5625
+NGC 2264 Sector KQ-R c19-0,NGC 2264 Sector,Unknown,579.9375,98.15625,-1700.6875
+NGC 2264 Sector KS-M b23-0,NGC 2264 Sector,Unknown,1098.34375,62.21875,-2012.09375
+NGC 2264 Sector KS-V b18-0,NGC 2264 Sector,Unknown,1020.5,80.5,-2121.375
+NGC 2264 Sector LC-C a58-0,NGC 2264 Sector,Unknown,567.5,-42.40625,-1902.46875
+NGC 2264 Sector LD-A b30-0,NGC 2264 Sector,Unknown,520.1875,38.71875,-1881.3125
+NGC 2264 Sector LE-O c7-1,NGC 2264 Sector,Unknown,488.46875,24.46875,-2214.4375
+NGC 2264 Sector LE-O c7-2,NGC 2264 Sector,Unknown,485.59375,22.15625,-2218.46875
+NGC 2264 Sector LE-O c7-3,NGC 2264 Sector,Unknown,492.09375,31.5625,-2199.9375
+NGC 2264 Sector LH-V e2-1,NGC 2264 Sector,Unknown,1133.9375,74.90625,-2109.09375
+NGC 2264 Sector LI-R c18-0,NGC 2264 Sector,Unknown,973.65625,-325.125,-1730.1875
+NGC 2264 Sector LO-C b15-0,NGC 2264 Sector,Unknown,795.71875,72.8125,-2189.5625
+NGC 2264 Sector LS-K b24-0,NGC 2264 Sector,Unknown,494.875,46.03125,-2001.15625
+NGC 2264 Sector LS-M b23-0,NGC 2264 Sector,Unknown,1120.25,59.4375,-2019.125
+NGC 2264 Sector MB-R a77-0,NGC 2264 Sector,Unknown,516.5,-44.03125,-1692.59375
+NGC 2264 Sector MF-L c22-1,NGC 2264 Sector,Unknown,761.25,-132.125,-1580.40625
+NGC 2264 Sector MI-A b43-0,NGC 2264 Sector,Unknown,741.21875,-76.625,-1597.78125
+NGC 2264 Sector ML-H b12-0,NGC 2264 Sector,Unknown,472.71875,21.8125,-2259.78125
+NGC 2264 Sector MO-Q d5-13,NGC 2264 Sector,Unknown,382.625,-4.6875,-2069.28125
+NGC 2264 Sector MS-M b23-0,NGC 2264 Sector,Unknown,1139.90625,56.34375,-2019.46875
+NGC 2264 Sector MT-Q d5-10,NGC 2264 Sector,Unknown,540.8125,65.90625,-2066.65625
+NGC 2264 Sector MV-B a72-0,NGC 2264 Sector,Unknown,521.71875,-34.4375,-1749.09375
+NGC 2264 Sector MX-S c4-1,NGC 2264 Sector,Unknown,752.0625,-71.4375,-2309.25
+NGC 2264 Sector MX-S c4-3,NGC 2264 Sector,Unknown,760.09375,-68.0625,-2343.53125
+NGC 2264 Sector NH-L c8-1,NGC 2264 Sector,Unknown,939.25,-217.8125,-2170.90625
+NGC 2264 Sector NH-X b17-0,NGC 2264 Sector,Unknown,1123.09375,71.5625,-2137.9375
+NGC 2264 Sector NJ-S b20-0,NGC 2264 Sector,Unknown,958.40625,97.34375,-2083.625
+NGC 2264 Sector NM-L b24-0,NGC 2264 Sector,Unknown,679.28125,115.96875,-2002.21875
+NGC 2264 Sector NO-H d10-5,NGC 2264 Sector,Unknown,756.96875,-68.4375,-1630.5
+NGC 2264 Sector NS-M b23-0,NGC 2264 Sector,Unknown,1158.75,60.40625,-2019
+NGC 2264 Sector NX-K d8-10,NGC 2264 Sector,Unknown,1250.3125,-145.0625,-1848.03125
+NGC 2264 Sector NX-K d8-16,NGC 2264 Sector,Unknown,1219.59375,-167.09375,-1810.59375
+NGC 2264 Sector NY-T b19-0,NGC 2264 Sector,Unknown,1000.46875,78.71875,-2100.875
+NGC 2264 Sector OH-V b18-0,NGC 2264 Sector,Unknown,498.1875,40,-2121.09375
+NGC 2264 Sector OI-B b16-0,NGC 2264 Sector,Unknown,381.3125,115.28125,-2169.53125
+NGC 2264 Sector OI-J d9-8,NGC 2264 Sector,Unknown,1212.0625,-87.1875,-1766.75
+NGC 2264 Sector OJ-Y b30-0,NGC 2264 Sector,Unknown,513.125,49.3125,-1845.4375
+NGC 2264 Sector OS-M b23-0,NGC 2264 Sector,Unknown,1187.53125,58.46875,-2005.84375
+NGC 2264 Sector OV-B c14-2,NGC 2264 Sector,Unknown,506.40625,40.90625,-1930.96875
+NGC 2264 Sector PB-A a73-0,NGC 2264 Sector,Unknown,514.875,-33.4375,-1740.1875
+NGC 2264 Sector PF-C b43-0,NGC 2264 Sector,Unknown,682.8125,127.6875,-1591.6875
+NGC 2264 Sector PJ-Y b30-0,NGC 2264 Sector,Unknown,519.125,38.875,-1863.84375
+NGC 2264 Sector PK-M c8-0,NGC 2264 Sector,Unknown,491.65625,34.28125,-2170.1875
+NGC 2264 Sector PN-M b23-0,NGC 2264 Sector,Unknown,1173.53125,52.25,-2010.375
+NGC 2264 Sector PP-D b42-0,NGC 2264 Sector,Unknown,675.6875,80.03125,-1619.59375
+NGC 2264 Sector PS-M b23-0,NGC 2264 Sector,Unknown,1203.375,60.09375,-2009.03125
+NGC 2264 Sector PY-I b25-0,NGC 2264 Sector,Unknown,485.875,41.4375,-1982
+NGC 2264 Sector PZ-O b22-0,NGC 2264 Sector,Unknown,358.78125,119.28125,-2044.21875
+NGC 2264 Sector QA-C b43-0,NGC 2264 Sector,Unknown,658.65625,99.03125,-1602.5625
+NGC 2264 Sector QB-A a73-0,NGC 2264 Sector,Unknown,518.3125,-32.3125,-1741.1875
+NGC 2264 Sector QE-S b20-0,NGC 2264 Sector,Unknown,979.65625,75.0625,-2084.03125
+NGC 2264 Sector QF-Z b16-0,NGC 2264 Sector,Unknown,787.03125,86.6875,-2163.71875
+NGC 2264 Sector QG-J c10-0,NGC 2264 Sector,Unknown,356.09375,124.5625,-2072.1875
+NGC 2264 Sector QG-J c10-1,NGC 2264 Sector,Unknown,353.34375,120.3125,-2097.25
+NGC 2264 Sector QR-F b13-0,NGC 2264 Sector,Unknown,471.09375,33.46875,-2227.78125
+NGC 2264 Sector QS-M b23-0,NGC 2264 Sector,Unknown,1219.5,56.9375,-2006.78125
+NGC 2264 Sector RF-L c22-1,NGC 2264 Sector,Unknown,961.03125,-121.5,-1561.1875
+NGC 2264 Sector RH-F b26-0,NGC 2264 Sector,Unknown,556.0625,-146.4375,-1958.78125
+NGC 2264 Sector RL-Q b7-0,NGC 2264 Sector,Unknown,478.9375,50.1875,-2349.375
+NGC 2264 Sector RN-C b41-0,NGC 2264 Sector,Unknown,718.78125,-220.75,-1644.0625
+NGC 2264 Sector RU-F d11-10,NGC 2264 Sector,Unknown,758.40625,-82.75,-1614.78125
+NGC 2264 Sector RU-O d6-14,NGC 2264 Sector,Unknown,484.75,31.28125,-1955.625
+NGC 2264 Sector SI-E b14-0,NGC 2264 Sector,Unknown,1018.46875,55.96875,-2222.75
+NGC 2264 Sector SJ-Y b43-0,NGC 2264 Sector,Unknown,735.15625,-91.28125,-1582.40625
+NGC 2264 Sector SN-J b25-0,NGC 2264 Sector,Unknown,672.5625,102.5,-1980.25
+NGC 2264 Sector SN-T b19-0,NGC 2264 Sector,Unknown,502.625,44,-2103.4375
+NGC 2264 Sector SP-F d11-0,NGC 2264 Sector,Unknown,722.15625,-127.46875,-1573.375
+NGC 2264 Sector SP-F d11-4,NGC 2264 Sector,Unknown,719.5,-109.5625,-1585.96875
+NGC 2264 Sector SY-Z b29-0,NGC 2264 Sector,Unknown,620.65625,18.25,-1879.3125
+NGC 2264 Sector SY-Z b42-0,NGC 2264 Sector,Unknown,776.15625,-122.3125,-1601.6875
+NGC 2264 Sector TB-S c18-1,NGC 2264 Sector,Unknown,537.65625,-183.53125,-1737.9375
+NGC 2264 Sector TE-H b26-0,NGC 2264 Sector,Unknown,478.875,38.75,-1962.96875
+NGC 2264 Sector TJ-Z b16-0,NGC 2264 Sector,Unknown,362.9375,106.625,-2148
+NGC 2264 Sector TT-K b24-0,NGC 2264 Sector,Unknown,1166.53125,41,-1999.84375
+NGC 2264 Sector TW-O b8-0,NGC 2264 Sector,Unknown,476.84375,60,-2340
+NGC 2264 Sector UA-H b39-0,NGC 2264 Sector,Unknown,1140.90625,-120.9375,-1680.40625
+NGC 2264 Sector UA-J b37-0,NGC 2264 Sector,Unknown,980,-281.40625,-1720.09375
+NGC 2264 Sector UD-D a85-0,NGC 2264 Sector,Unknown,563.25,-29.96875,-1605.4375
+NGC 2264 Sector UG-A b44-0,NGC 2264 Sector,Unknown,655.65625,97.15625,-1579.40625
+NGC 2264 Sector UH-Y a73-0,NGC 2264 Sector,Unknown,515.1875,-33.46875,-1733.5
+NGC 2264 Sector UL-K b38-0,NGC 2264 Sector,Unknown,539.0625,57.4375,-1703.4375
+NGC 2264 Sector US-D b27-0,NGC 2264 Sector,Unknown,579,-142.28125,-1939.46875
+NGC 2264 Sector UU-F d11-0,NGC 2264 Sector,Unknown,985.25,-92.75,-1624.9375
+NGC 2264 Sector VA-J b37-0,NGC 2264 Sector,Unknown,1008.0625,-277.875,-1713.28125
+NGC 2264 Sector VA-V b32-0,NGC 2264 Sector,Unknown,519.875,55.96875,-1824.28125
+NGC 2264 Sector VB-J b39-0,NGC 2264 Sector,Unknown,568.71875,112.1875,-1684.28125
+NGC 2264 Sector VF-Q b21-0,NGC 2264 Sector,Unknown,959.1875,58.40625,-2061.1875
+NGC 2264 Sector VO-F c12-1,NGC 2264 Sector,Unknown,1178.46875,56.59375,-2021.90625
+NGC 2264 Sector VP-J b11-0,NGC 2264 Sector,Unknown,800.6875,67.4375,-2280.625
+NGC 2264 Sector VV-I b37-0,NGC 2264 Sector,Unknown,971.75,-302.9375,-1718.625
+NGC 2264 Sector VX-D b14-0,NGC 2264 Sector,Unknown,483.3125,27.65625,-2217.125
+NGC 2264 Sector VY-K b24-0,NGC 2264 Sector,Unknown,1242.71875,59.59375,-2000.1875
+NGC 2264 Sector VY-T b19-0,NGC 2264 Sector,Unknown,1160.03125,80.6875,-2099.40625
+NGC 2264 Sector VZ-G b26-0,NGC 2264 Sector,Unknown,490.6875,25.28125,-1948.21875
+NGC 2264 Sector VZ-X b43-0,NGC 2264 Sector,Unknown,717.84375,-144.90625,-1580.5625
+NGC 2264 Sector WK-O d6-1,NGC 2264 Sector,Unknown,537.34375,-158.5625,-1980.78125
+NGC 2264 Sector WP-F d11-13,NGC 2264 Sector,Unknown,990.625,-105.78125,-1601.9375
+NGC 2264 Sector WP-V c3-2,NGC 2264 Sector,Unknown,693.625,130.65625,-2348.28125
+NGC 2264 Sector WT-R b20-0,NGC 2264 Sector,Unknown,497.46875,36.5625,-2084.125
+NGC 2264 Sector WY-C a85-0,NGC 2264 Sector,Unknown,557,-39.8125,-1611.625
+NGC 2264 Sector WY-K b24-0,NGC 2264 Sector,Unknown,1256.4375,55.90625,-1999.96875
+NGC 2264 Sector WY-T b19-0,NGC 2264 Sector,Unknown,1183.5625,78.53125,-2088.28125
+NGC 2264 Sector WZ-G d10-5,NGC 2264 Sector,Unknown,1013.1875,-273.21875,-1684.1875
+NGC 2264 Sector XA-L c9-1,NGC 2264 Sector,Unknown,785.1875,97.96875,-2134.8125
+NGC 2264 Sector XC-N b9-0,NGC 2264 Sector,Unknown,478.78125,57.34375,-2319.09375
+NGC 2264 Sector XM-Y b44-0,NGC 2264 Sector,Unknown,641.15625,96.5,-1551.6875
+NGC 2264 Sector XN-W a74-0,NGC 2264 Sector,Unknown,514.90625,-33.34375,-1717.25
+NGC 2264 Sector XQ-T c4-0,NGC 2264 Sector,Unknown,476.4375,56.78125,-2312.0625
+NGC 2264 Sector XX-E b42-0,NGC 2264 Sector,Unknown,597.40625,217.84375,-1623.78125
+NGC 2264 Sector XY-K b24-0,NGC 2264 Sector,Unknown,1276.21875,59.6875,-1999.625
+NGC 2264 Sector YG-L b24-0,NGC 2264 Sector,Unknown,346.8125,107,-1993.28125
+NGC 2264 Sector YH-X c16-0,NGC 2264 Sector,Unknown,756.15625,183.15625,-1814.5625
+NGC 2264 Sector YI-C b15-0,NGC 2264 Sector,Unknown,495.9375,36.78125,-2191.71875
+NGC 2264 Sector YK-F b27-0,NGC 2264 Sector,Unknown,496.78125,35.75,-1939.125
+NGC 2264 Sector YN-W a74-0,NGC 2264 Sector,Unknown,517.09375,-34.125,-1722.09375
+NGC 2264 Sector YS-F c12-1,NGC 2264 Sector,Unknown,356.5,121.46875,-2014.84375
+NGC 2264 Sector YW-I c10-0,NGC 2264 Sector,Unknown,497.59375,32.53125,-2093.5625
+NGC 2264 Sector ZD-L c23-0,NGC 2264 Sector,Unknown,777.25,220,-1544.625
+NGC 2264 Sector ZO-D b1-0,NGC 2264 Sector,Unknown,838.15625,120.4375,-2500.15625
+NGC 2264 Sector ZT-K b24-0,NGC 2264 Sector,Unknown,1294.34375,47.375,-2003.9375
+NGC 2264 Sector ZY-B b28-0,NGC 2264 Sector,Unknown,599.15625,-141.84375,-1923.90625
+NGC 2286 Sector AE-J b12-0,NGC 2264 Sector,Unknown,5308.8125,-112.75,-7827.375
+NGC 2286 Sector GS-S d4-30,NGC 2264 Sector,Unknown,5259.65625,-120,-7686.5625
+NGC 2286 Sector WD-T d3-1,NGC 2264 Sector,Unknown,5695.53125,-674,-7754.625
+NGC 3603 Sector GW-W d1-44,NGC 3603 Sector,Unknown,18614.875,-173.3125,7370.25
+NGC 3603 Sector MX-U d2-16,NGC 3603 Sector,Unknown,18653.4375,-189.0625,7432.78125
diff --git a/data/csvCache/expedition.csv b/data/csvCache/expedition.csv
new file mode 100644
index 0000000..f131d8a
--- /dev/null
+++ b/data/csvCache/expedition.csv
@@ -0,0 +1,125 @@
+name,pos_x,pos_y,pos_z,infos
+HIP 15310,-10.75000,-87.00000,-104.00000,Proto-Lagrange Cloud
Purpureum Metallic Crystals
Rubeum Metallic Crystals
Flavum Metallic Crystals
Q04-Type Anomaly
Q08-Type Anomaly
+Alaunus,-0.65625,-52.00000,-5.96875,Proto-Lagrange Cloud
Solid Mineral Spheres
Purpureum Metallic Crystals
Rubeum Metallic Crystals
Flavum Metallic Crystals
Q09-Type Anomaly
+Col 285 Sector GG-N c7-34,181.00000,4.40625,-41.00000,Proto-Lagrange Cloud
Prasinum Metallic Crystals
Purpureum Metallic Crystals
Rubeum Metallic Crystals
Flavum Metallic Crystals
Croceum Gourd Mollusc
+116 Tauri,67.34375,-72.34375,-412.46875,Sulphur Dioxide Fumarole
Sulphur Dioxide Gas Vent
Luteolum Anemone
Croceum Anemone
Blatteum Bioluminescent Anemone
Rubeum Bioluminescent Anemone
+Oochorrs NF-L d9-12,216.00000,-55.00000,-1490.96875,Carbon Dioxide Ice Fumarole
Carbon Dioxide Ice Geyser
Crystalline Shards
+BD-12 1172,578.00000,-452.68750,-819.00000,Silicate Vapour Fumarole
Silicate Magma Lava Spout
Iron Magma Lava Spout
Sulphur Dioxide Gas Vent
Silicate Vapour Gas Vent
Solid Mineral Spheres
Lattice Mineral Spheres
Prasinum Bioluminescent Anemone
Rubeum Metallic Crystals
Flavum Metallic Crystals
Viride Gourd Mollusc
+Col 135 Sector DR-V c2-16,818.06250,-169.21875,-407.37500,Water Ice Fumarole
Viride Brain Tree
+HD 63276,1083.68750,-250.53125,-102.62500,Sulphur Dioxide Gas Vent
Roseum Brain Tree
Lividum Brain Tree
Aureum Brain Tree
Lindigoticum Brain Tree
+HD 81946,1428.68750,36.62500,62.40625,Sulphur Dioxide Fumarole
Silicate Magma Lava Spout
Silicate Vapour Gas Vent
Proto-Lagrange Cloud
Roseum Brain Tree
Ostrinum Brain Tree
Lividum Brain Tree
Aureum Brain Tree
Roseum Bioluminescent Anemone
Prasinum Metallic Crystals
Rubeum Metallic Crystals
Albulum Gourd Mollusc
+Eta Carina Sector JH-V c2-9,8606.00000,-122.00000,2705.00000,Croceum Lagrange Cloud
Bark Mounds
Lindigoticum Ice Crystals
Flavum Ice Crystals
Flavum Silicate Crystals
Rubeum Metallic Crystals
Luteolum Bell Mollusc
+Eta Carina Sector EL-Y d19,8625.34375,-159.75000,2694.00000,Silicate Vapour Fumarole
Silicate Vapour Gas Vent
Viride Lagrange Cloud
Luteolum Lagrange Cloud
Solid Mineral Spheres
Roseum Brain Tree
Gypseeum Brain Tree
Lividum Brain Tree
Aureum Brain Tree
Bark Mounds
Lindigoticum Silicate Crystals
Roseum Silicate Crystals
Albens Bell Mollusc
+GCRV 6493,8798.00000,-785.84375,2573.00000,Roseum Lagrange Cloud
Prasinum Metallic Crystals
Flavum Metallic Crystals
P04-Type Anomaly
+Thaile HW-V e2-7,10124.09375,-339.00000,4641.09375,Sulphur Dioxide Fumarole
Sulphur Dioxide Gas Vent
Croceum Lagrange Cloud
Croceum Lagrange Storm Cloud
Albidum Peduncle Tree
L06-Type Anomaly
+Drokoe AN-H d11-6,14556.03125,-169.18750,3785.68750,Proto-Lagrange Cloud
Roseum Ice Crystals
Albidum Ice Crystals
Rubeum Silicate Crystals
Albidum Silicate Crystals
Purpureum Metallic Crystals
Rubeum Metallic Crystals
Cereum Bullet Mollusc
Viride Bullet Mollusc
+Drokoe FU-O b39-0,14600.21875,-160.15625,3619.31250,Solid Mineral Spheres
Lindigoticum Silicate Crystals
Prasinum Metallic Crystals
Rubeum Metallic Crystals
Flavum Metallic Crystals
Cereum Bullet Mollusc
Rubeum Bullet Mollusc
Guardian Codex
Guardian Relic Tower
+Drokoe QK-X b34-0,14660.56250,-183.43750,3517.25000,Prasinum Ice Crystals
Roseum Ice Crystals
Flavum Bullet Mollusc
+NGC 3199 Sector EB-X c1-6,14558.00000,-252.00000,3511.28125,Viride Lagrange Cloud
Roseum Lagrange Cloud
Rubicundum Lagrange Cloud
Croceum Lagrange Cloud
Bark Mounds
Lindigoticum Ice Crystals
Prasinum Ice Crystals
Rubeum Ice Crystals
Purpureum Metallic Crystals
Rubeum Metallic Crystals
Lividum Bullet Mollusc
Viride Bullet Mollusc
+Lyed XJ-I d9-0,10938.87500,35.37500,-16845.28125,Proto-Lagrange Cloud
Viride Gyre Tree
P07-Type Anomaly
+Lyed YJ-I d9-0,11007.46875,44.84375,-16899.75000,Proto-Lagrange Cloud
Viride Gyre Tree
P05-Type Anomaly
+Eorgh Hypa RR-U c19-0,17339.00000,-99.00000,-13023.53125,Sulphur Dioxide Fumarole
Silicate Vapour Fumarole
Silicate Magma Lava Spout
Iron Magma Lava Spout
Sulphur Dioxide Gas Vent
Silicate Vapour Gas Vent
Proto-Lagrange Cloud
Roseum Gyre Pod
Viride Gyre Tree
+Jongoae UX-L d7-0,25387.06250,-20.21875,-9381.43750,Proto-Lagrange Cloud
Lindigoticum Ice Crystals
Aurarium Gyre Pod
Viride Gyre Tree
Aurarium Gyre Tree
+Hyphaups HC-M d7-1,36077.34375,94.81250,8597.46875,Proto-Lagrange Cloud
Roseum Silicate Crystals
Purpureum Silicate Crystals
Rubeum Silicate Crystals
Rubeum Rhizome Pod
+Hyphaups NI-K d8-0,36187.15625,55.31250,8624.40625,Rubeum Ice Crystals
Purpureum Rhizome Pod
+Oodgosly AM-D d12-7,34869.15625,159.65625,11556.25000,Proto-Lagrange Cloud
Roseum Ice Crystals
Flavum Ice Crystals
Blatteum Quadripartite Pod
+Oodgosly AH-D d12-10,34733.75000,105.84375,11526.21875,Prasinum Ice Crystals
Roseum Ice Crystals
Rubeum Ice Crystals
Roseum Silicate Crystals
Albidum Quadripartite Pod
Caeruleum Quadripartite Pod
+Oodgosly GI-B d13-11,34678.00000,42.00000,11620.00000,Iron Magma Lava Spout
Lindigoticum Ice Crystals
Roseum Ice Crystals
Purpureum Ice Crystals
Rubeum Ice Crystals
Flavum Ice Crystals
Lindigoticum Silicate Crystals
Viride Quadripartite Pod
+Coesky DW-U d3-0,35056.59375,-498.75000,15903.43750,Purpureum Ice Crystals
Flavum Ice Crystals
Prasinum Silicate Crystals
Candidum Rhizome Pod
Gypseeum Rhizome Pod
+Ood Fleau ZJ-I d9-0,40504.00000,26.00000,17678.00000,Proto-Lagrange Cloud
Lindigoticum Ice Crystals
Lindigoticum Silicate Crystals
L08-Type Anomaly
+Dryeou Fleau XJ-A d0,39744.59375,168.84375,18188.71875,Roseum Ice Crystals
Flavum Ice Crystals
Prasinum Silicate Crystals
Purpureum Silicate Crystals
Rubeum Silicate Crystals
Candidum Rhizome Pod
Cobalteum Rhizome Pod
+Braisao ZP-V d3-16,25166.53125,-160.53125,31257.90625,Proto-Lagrange Cloud
Candidum Peduncle Pod
Caeruleum Peduncle Pod
Gypseeum Peduncle Pod
Caeruleum Peduncle Tree
Viride Peduncle Tree
Ostrinum Peduncle Tree
Rubellum Peduncle Tree
+Phreia Byio PU-K c10-0,23577.06250,-421.12500,36500.78125,Proto-Lagrange Cloud
Purpureum Metallic Crystals
Candidum Peduncle Pod
Purpureum Peduncle Pod
Rufum Peduncle Pod
Caeruleum Peduncle Tree
Viride Peduncle Tree
+Joorai NI-K d8-9,16980.84375,75.81250,39353.75000,Proto-Lagrange Cloud
Purpureum Metallic Crystals
Rubeum Metallic Crystals
Flavum Metallic Crystals
P08-Type Anomaly
P09-Type Anomaly
+Joorai NI-K d8-115,17016.46875,106.53125,39349.90625,Proto-Lagrange Cloud
Purpureum Metallic Crystals
Rubeum Metallic Crystals
Flavum Metallic Crystals
P14-Type Anomaly
+Joorai NI-K d8-163,17052.00000,117.06250,39384.00000,Proto-Lagrange Cloud
Purpureum Metallic Crystals
Rubeum Metallic Crystals
P12-Type Anomaly
+Plua Chruia IB-X d1-5,22205.78125,231.75000,47756.28125,Proto-Lagrange Cloud
Lindigoticum Silicate Crystals
Albidum Chalice Pod
+Syrivu DL-P d5-2,15750.00000,-8.81250,54475.40625,Albidum Ice Crystals
Flavum Ice Crystals
Ostrinum Chalice Pod
+Ploea Brou BL-Y b14-5,6009.00000,42.00000,50474.37500,Roseum Ice Crystals
Flavum Ice Crystals
Rubeum Squid Mollusc
+Preae Chroa EI-I c23-43,5572.18750,83.34375,49885.53125,Prasinum Ice Crystals
Albidum Ice Crystals
Roseum Squid Mollusc
+Flyeia Byoea UK-Q b24-6,5550.00000,-221.84375,50662.37500,Flavum Ice Crystals
Puniceum Squid Mollusc
+Flyeia Byoea GE-K c11-32,5626.00000,-174.00000,50641.00000,Rubeum Ice Crystals
Purpureum Silicate Crystals
Albulum Squid Mollusc
+Ploea Brou RH-D c12-27,5827.00000,29.09375,50671.00000,Rubeum Ice Crystals
Lindigoticum Silicate Crystals
Caeruleum Squid Mollusc
+Pyra Dryoae ET-O d7-7,7825.40625,-101.96875,62316.93750,Proto-Lagrange Cloud
Rubeum Ice Crystals
Caeruleum Chalice Pod
+Iorady EI-B d13-0,-0.31250,-3.59375,65395.28125,Proto-Lagrange Cloud
Lindigoticum Silicate Crystals
K13-Type Anomaly
+Pyrooe Dryiae CC-B d1-0,-5017.87500,-97.00000,61738.00000,Proto-Lagrange Cloud
Cereum Aster Pod
Cereum Aster Tree
+Pyrooe Dryiae ZQ-C d0,-5048.68750,-105.71875,61687.34375,Lindigoticum Ice Crystals
Flavum Ice Crystals
Prasinum Silicate Crystals
Roseum Silicate Crystals
Puniceum Aster Pod
+Cliewoae DS-H d11-0,-5574.00000,-103.00000,61347.00000,Proto-Lagrange Cloud
Rubellum Aster Pod
Rubellum Aster Tree
+Cliewoae UF-L d9-0,-5629.09375,-63.62500,61189.40625,Prasinum Aster Pod
Prasinum Aster Tree
+Cliewoae VA-L d9-0,-5733.00000,-176.62500,61178.18750,Proto-Lagrange Cloud
Purpureum Ice Crystals
Rubeum Ice Crystals
Albidum Ice Crystals
Flavum Silicate Crystals
Lindigoticum Aster Pod
+Flyoo Groa SO-Z e0,-26482.00000,-78.78125,50335.12500,Roseum Lagrange Cloud
Stolon Pod
Stolon Tree
+Aiphaisty YE-A d130,-17942.84375,102.84375,33508.75000,Silicate Vapour Fumarole
Prasinum Metallic Crystals
Flavum Metallic Crystals
Q07-Type Anomaly
+Dryu Chraea FH-D d12-49,-23799.71875,71.12500,29480.12500,Proto-Lagrange Cloud
Luteolum Calcite Plates
Lindigoticum Calcite Plates
Viride Calcite Plates
Q01-Type Anomaly
+Phooe Auf HV-I c24-0,-15057.25000,1832.87500,26889.65625,Purpureum Metallic Crystals
Flavum Metallic Crystals
E01-Type Anomaly
+Wembeau KM-V e2-12,-16947.00000,-529.65625,23808.46875,Water Ice Geyser
Prasinum Metallic Crystals
Purpureum Metallic Crystals
L03-Type Anomaly
+Byaa Thoi MI-B d13-0,-35234.00000,26.46875,3917.00000,K07-Type Anomaly
+Cyuefoo LC-D d12-0,-33742.96875,53.50000,26.46875,Proto-Lagrange Cloud
Lindigoticum Silicate Crystals
L09-Type Anomaly
+Ovomly DA-Q d5-16,-19614.78125,-1012.59375,7113.00000,Proto-Lagrange Cloud
Rubeum Metallic Crystals
Luteolum Calcite Plates
Lindigoticum Calcite Plates
Rubellum Torus Mollusc
+Ovomly HG-O d6-0,-19610.00000,-1000.65625,7217.46875,Water Ice Geyser
Proto-Lagrange Cloud
Luteolum Calcite Plates
Lindigoticum Calcite Plates
Viride Calcite Plates
Rutulum Calcite Plates
Blatteum Torus Mollusc
+Ovomly SS-K d8-8,-19411.84375,-1036.15625,7345.15625,Luteolum Calcite Plates
Rutulum Calcite Plates
Viride Torus Mollusc
+Ovomly AA-H d10-13,-19537.25000,-1114.40625,7564.09375,Lattice Mineral Spheres
Prasinum Metallic Crystals
Purpureum Metallic Crystals
Rutulum Calcite Plates
Caeruleum Torus Mollusc
+Prai Hypoo CY-C b3-1,-9287.96875,-432.31250,7962.75000,Iron Magma Lava Spout
Croceum Lagrange Cloud
Albidum Peduncle Tree
Blatteum Bell Mollusc
+Traikeou SE-P d6-16,-7188.81250,683.18750,4626.00000,Viride Lagrange Cloud
Croceum Lagrange Cloud
Bark Mounds
Rubeum Metallic Crystals
Flavum Metallic Crystals
Purpureum Gourd Mollusc
+Ellaisms QX-U e2-43,-5469.93750,260.09375,9674.00000,Sulphur Dioxide Fumarole
Iron Magma Lava Spout
Solid Mineral Spheres
P02-Type Anomaly
+Skaudai YP-O e6-17,-5485.00000,-503.00000,10416.00000,Sulphur Dioxide Fumarole
Viride Lagrange Cloud
Luteolum Lagrange Cloud
Croceum Lagrange Cloud
Proto-Lagrange Cloud
Solid Mineral Spheres
Lattice Mineral Spheres
Purpureum Metallic Crystals
Rubeum Metallic Crystals
Flavum Metallic Crystals
Gypseeum Bell Mollusc
+Dryoea Flyi II-S e4-6870,-5141.59375,-289.46875,19057.90625,Luteolum Lagrange Storm Cloud
Solid Mineral Spheres
Blatteum Sinuous Tubers
Lindigoticum Sinuous Tubers
Violaceum Sinuous Tubers
Viride Sinuous Tubers
Prasinum Metallic Crystals
Rubeum Metallic Crystals
Flavum Metallic Crystals
K12-Type Anomaly
+Eol Flyou KC-V f2-5802,-9481.84375,-534.53125,17880.31250,Purpureum Metallic Crystals
Rubeum Metallic Crystals
Flavum Metallic Crystals
Croceum Globe Mollusc
+Eol Flyou DL-Y g117,-9473.53125,-498.40625,17879.90625,Solid Mineral Spheres
Prasinum Metallic Crystals
Purpureum Metallic Crystals
Rubeum Metallic Crystals
Cobalteum Globe Mollusc
+Eol Flyou KC-V f2-266,-9492.78125,-479.37500,17860.03125,Solid Mineral Spheres
Prasinum Bioluminescent Anemone
Prasinum Metallic Crystals
Niveum Globe Mollusc
Rutulum Globe Mollusc
+Eol Flyou KC-V f2-3149,-9523.43750,-504.59375,17873.37500,Proto-Lagrange Cloud
Solid Mineral Spheres
Purpureum Metallic Crystals
Roseum Globe Mollusc
+Eol Flyou KC-V f2-1398,-9520.56250,-491.21875,17893.06250,Prasinum Globe Mollusc
+Eol Flyou KC-V f2-5570,-9507.25000,-485.81250,17949.25000,Proto-Lagrange Cloud
Prasinum Metallic Crystals
Ostrinum Globe Mollusc
+Eol Prou IW-W e1-1868,-9530.00000,-956.00000,19790.00000,Sulphur Dioxide Gas Vent
Caeruleum Lagrange Cloud
Purpureum Metallic Crystals
Rubeum Metallic Crystals
Lindigoticum Umbrella Mollusc
+Asura,-9550.00000,-916.65625,19816.18750,Sulphur Dioxide Fumarole
Viride Lagrange Cloud
Roseum Lagrange Cloud
Solid Mineral Spheres
Prasinum Metallic Crystals
Flavum Metallic Crystals
Luteolum Umbrella Mollusc
Viride Umbrella Mollusc
+Wepaa BF-A f494,-9287.62500,-875.90625,23438.53125,Caeruleum Lagrange Cloud
Croceum Lagrange Cloud
Solid Mineral Spheres
Prasinum Metallic Crystals
Rubeum Metallic Crystals
K01-Type Anomaly
K03-Type Anomaly
+Wepaa GG-Y f343,-9484.21875,-1071.59375,23759.43750,Proto-Lagrange Cloud
Prasinum Metallic Crystals
Rubeum Metallic Crystals
Flavum Metallic Crystals
K04-Type Anomaly
K06-Type Anomaly
K08-Type Anomaly
+Wepaa BA-A g524,-9308.71875,-708.65625,23829.75000,Roseum Lagrange Cloud
Croceum Lagrange Cloud
Solid Mineral Spheres
Prasinum Metallic Crystals
K05-Type Anomaly
E04-Type Anomaly
+Dryio Bloo LT-Y d1-311,-6377.37500,-1587.09375,28545.87500,Caeruleum Lagrange Cloud
Solid Mineral Spheres
Prasinum Metallic Crystals
Rubeum Metallic Crystals
Flavum Metallic Crystals
Luteolum Capsule Mollusc
+Dryio Bloo LT-Y d1-1089,-6338.12500,-1561.40625,28548.28125,Silicate Vapour Fumarole
Viride Lagrange Cloud
Bark Mounds
Prasinum Metallic Crystals
Flavum Metallic Crystals
Viride Capsule Mollusc
+Dryio Bloo YE-A g1518,-6353.50000,-1620.75000,28610.78125,Rubicundum Lagrange Cloud
Purpureum Metallic Crystals
Flavum Metallic Crystals
Lindigoticum Capsule Mollusc
+Xothuia EG-Y g95,-7695.00000,607.21875,30656.00000,Viride Lagrange Cloud
Rubicundum Lagrange Cloud
Croceum Lagrange Cloud
Solid Mineral Spheres
Prasinum Metallic Crystals
Lindigoticum Bulb Mollusc
+Eorl Bre TE-L c23-51,-7754.78125,690.00000,30685.03125,Luteolum Lagrange Cloud
Roseum Lagrange Cloud
Purpureum Metallic Crystals
Rubeum Metallic Crystals
Flavum Metallic Crystals
Viride Bulb Mollusc
+Eorl Bre ZP-E d12-276,-7747.00000,686.34375,30699.00000,Caeruleum Lagrange Cloud
Rubicundum Lagrange Cloud
Purpureum Metallic Crystals
Rubeum Metallic Crystals
Flavum Metallic Crystals
Luteolum Bulb Mollusc
+Phleedgaa JS-I d10-280,-5360.46875,-418.90625,34447.87500,Caeruleum Lagrange Cloud
Croceum Lagrange Cloud
Solid Mineral Spheres
Purpureum Metallic Crystals
Rubeum Metallic Crystals
E03-Type Anomaly
+Phleedgaa UJ-Q e5-185,-5370.06250,-467.00000,34479.03125,Luteolum Lagrange Cloud
Roseum Lagrange Storm Cloud
Solid Mineral Spheres
Rubeum Metallic Crystals
E03-Type Anomaly
+Vegnue UE-Q e5-33,-5705.00000,624.21875,37122.40625,Caeruleum Lagrange Cloud
Viride Lagrange Cloud
Rubicundum Lagrange Cloud
Croceum Lagrange Cloud
Solid Mineral Spheres
Prasinum Metallic Crystals
Purpureum Metallic Crystals
Luteolum Parasol Mollusc
Viride Parasol Mollusc
+Vegnue AG-O e6-199,-5688.00000,547.25000,37182.00000,Solid Mineral Spheres
Lattice Mineral Spheres
Purpureum Metallic Crystals
Lindigoticum Parasol Mollusc
+Vegnoae QO-I d9-2277,-1008.03125,106.65625,36872.46875,Rubicundum Lagrange Cloud
Croceum Lagrange Cloud
Flavum Metallic Crystals
E02-Type Anomaly
+Phraa Pra HH-U e3-1354,823.31250,-1416.00000,26584.43750,Viride Lagrange Storm Cloud
Solid Mineral Spheres
Flavum Metallic Crystals
T02-Type Anomaly
+Juenae OX-U e2-8852,652.46875,-1056.50000,26400.37500,Rubicundum Lagrange Cloud
Prasinum Metallic Crystals
Rubeum Metallic Crystals
L01-Type Anomaly
+Stuemeae FG-Y d7561,29.00000,-19.78125,25900.00000,Proto-Lagrange Cloud
Prasinum Metallic Crystals
Purpureum Metallic Crystals
L04-Type Anomaly
+Stuemeae KM-W c1-342,26.34375,-20.56250,25899.25000,Water Ice Fumarole
Water Ice Geyser
Solid Mineral Spheres
Prasinum Metallic Crystals
K11-Type Anomaly
+Nyuena RO-Z d184,29.46875,1831.00000,25900.31250,Silicate Magma Lava Spout
Proto-Lagrange Cloud
Purpureum Metallic Crystals
Rubeum Metallic Crystals
Flavum Metallic Crystals
P15-Type Anomaly
+Nyuena ID-Z c1-10,29.96875,1837.75000,25897.43750,Proto-Lagrange Cloud
Prasinum Metallic Crystals
Purpureum Metallic Crystals
Flavum Metallic Crystals
P13-Type Anomaly
+Myriesly MS-T e3-3831,-586.87500,647.00000,25215.00000,Viride Lagrange Cloud
Rubicundum Lagrange Storm Cloud
Croceum Lagrange Cloud
Solid Mineral Spheres
Flavum Metallic Crystals
T04-Type Anomaly
+Myriesly CB-F d11-2373,-630.43750,116.25000,25562.78125,Solid Mineral Spheres
Purpureum Metallic Crystals
Q06-Type Anomaly
+Myriesly HR-N e6-4354,-530.00000,39.00000,25726.00000,Viride Lagrange Storm Cloud
Solid Mineral Spheres
Q02-Type Anomaly
+Byoomao MI-S e4-5423,-699.00000,-239.00000,25402.09375,Solid Mineral Spheres
Rubeum Metallic Crystals
Flavum Metallic Crystals
L05-Type Anomaly
+Byoomao JC-B d1-3681,-592.46875,-58.65625,24669.87500,Green Class III Gas Giant
Silicate Vapour Fumarole
Silicate Magma Lava Spout
Silicate Vapour Gas Vent
Proto-Lagrange Cloud
Prasinum Metallic Crystals
Purpureum Metallic Crystals
Rubeum Metallic Crystals
K10-Type Anomaly
+Agnairy JH-U e3-2113,-2713.65625,-77.00000,22618.00000,Water Ice Fumarole
Viride Lagrange Storm Cloud
Solid Mineral Spheres
Rubeum Metallic Crystals
T01-Type Anomaly
+Kyli Flyuae AA-A h4,-1683.93750,-3381.37500,23615.12500,Proto-Lagrange Cloud
Prasinum Metallic Crystals
Rubeum Metallic Crystals
Flavum Metallic Crystals
Q03-Type Anomaly
+Shrogeau GG-Y e119,5431.00000,32.00000,20932.06250,Rubicundum Lagrange Cloud
Rubicundum Lagrange Storm Cloud
Solid Mineral Spheres
Prasinum Metallic Crystals
Flavum Metallic Crystals
P03-Type Anomaly
+Shrogaae KK-A d2672,4952.90625,186.50000,20736.18750,Roseum Brain Tree
Lividum Brain Tree
Rubeum Octahedral Pod
Chryseum Void Heart
+Shrogaae KK-A d983,4959.03125,150.00000,20726.00000,Sulphur Dioxide Fumarole
Sulphur Dioxide Gas Vent
Roseum Brain Tree
Niveum Octahedral Pod
Chryseum Void Heart
+Shrogaae KK-A d1049,4945.06250,143.43750,20719.43750,Roseum Brain Tree
Viride Octahedral Pod
Chryseum Void Heart
+Shrogaae KK-A d1791,4957.34375,135.31250,20705.00000,Rubeum Metallic Crystals
Caeruleum Octahedral Pod
Chryseum Void Heart
+Gru Phio DV-W d2-440,3581.71875,-391.21875,15834.84375,Sulphur Dioxide Gas Vent
Roseum Sinuous Tubers
Prasinum Sinuous Tubers
Albidum Sinuous Tubers
Caeruleum Sinuous Tubers
+Eeshorks WO-A e191,1569.06250,-756.00000,16937.21875,Viride Lagrange Cloud
Luteolum Lagrange Cloud
Rubicundum Lagrange Cloud
Solid Mineral Spheres
Prasinum Metallic Crystals
Flavum Metallic Crystals
Viride Reel Mollusc
+Eeshorks QI-B d1496,1539.96875,-724.78125,16896.71875,Roseum Lagrange Cloud
Croceum Lagrange Cloud
Prasinum Metallic Crystals
Purpureum Metallic Crystals
Rubeum Metallic Crystals
Flavum Metallic Crystals
Luteolum Reel Mollusc
+Greae Phio DT-G d11-1570,1317.15625,-483.62500,16570.21875,Caeruleum Lagrange Cloud
Viride Lagrange Cloud
Solid Mineral Spheres
Purpureum Metallic Crystals
Lindigoticum Reel Mollusc
+Blu Ain QC-M d7-3330,913.28125,69.12500,14983.53125,Prasinum Metallic Crystals
Flavum Metallic Crystals
P10-Type Anomaly
+Gru Hypai DL-X e1-20,3384.12500,-364.00000,13444.43750,Solid Mineral Spheres
Prasinum Metallic Crystals
K02-Type Anomaly
+Blaa Hypai OZ-O d6-16,1286.00000,-731.65625,12309.34375,Viride Lagrange Cloud
Solid Mineral Spheres
Bark Mounds
Purpureum Metallic Crystals
Rubeum Metallic Crystals
Flavum Metallic Crystals
Lindigoticum Bell Mollusc
Guardian Codex
Guardian Relic Tower
+Graea Hypue DC-T d4-84,-1059.37500,-438.00000,13482.59375,Iron Magma Lava Spout
Sulphur Dioxide Gas Vent
Roseum Brain Tree
Gypseeum Brain Tree
Puniceum Brain Tree
Amphora Plant
+Eodgorsts TX-C b13-3,-1466.18750,141.59375,10743.43750,Prasinum Metallic Crystals
Flavum Metallic Crystals
Q05-Type Anomaly
+Pru Aescs NC-M d7-192,-3183.53125,74.00000,8596.00000,Sulphur Dioxide Fumarole
Silicate Vapour Fumarole
Silicate Magma Lava Spout
Sulphur Dioxide Gas Vent
Silicate Vapour Gas Vent
Purpureum Metallic Crystals
Flavum Metallic Crystals
P01-Type Anomaly
+Nyeajaae DA-Z a27-2,-3272.46875,-34.53125,6906.31250,Sulphur Dioxide Fumarole
Blatteum Sinuous Tubers
Lindigoticum Sinuous Tubers
Violaceum Sinuous Tubers
Viride Sinuous Tubers
+Trifid Sector BQ-Y d244,-641.00000,-16.00000,5168.00000,Viride Lagrange Cloud
Flavum Metallic Crystals
Blatteum Collared Pod
+Trifid Sector FW-W d1-233,-632.96875,-22.96875,5175.15625,Sulphur Dioxide Gas Vent
Bark Mounds
Lividum Collared Pod
+Trifid Sector DL-Y d157,-629.65625,-31.00000,5160.46875,Viride Lagrange Cloud
Roseum Lagrange Cloud
Rubicundum Lagrange Cloud
Solid Mineral Spheres
Prasinum Metallic Crystals
Purpureum Metallic Crystals
Flavum Metallic Crystals
Albidum Collared Pod
Rubicundum Collared Pod
+HD 160167,103.46875,-22.00000,1658.00000,Proto-Lagrange Cloud
Solid Mineral Spheres
Prasinum Metallic Crystals
Rubeum Metallic Crystals
Caeruleum Gourd Mollusc
+HIP 98182,-545.90625,7.09375,223.18750,Proto-Lagrange Cloud
Luteolum Anemone
Rubeum Metallic Crystals
Flavum Metallic Crystals
Rufum Gourd Mollusc
+HIP 115991,-409.03125,-317.90625,-69.81250,Silicate Vapour Fumarole
Silicate Magma Lava Spout
Roseum Anemone
Prasinum Metallic Crystals
Rubeum Metallic Crystals
Albulum Gourd Mollusc
+HIP 139,-1194.28125,126.53125,-648.21875,Puniceum Anemone
Prasinum Bioluminescent Anemone
+Wredguia XD-K d8-24,-249.78125,-8.93750,-301.96875,Sulphur Dioxide Fumarole
Silicate Magma Lava Spout
Sulphur Dioxide Gas Vent
Proto-Lagrange Cloud
Prasinum Metallic Crystals
Rubeum Metallic Crystals
Flavum Metallic Crystals
Phoeniceum Gourd Mollusc
+Varati,-178.65625,77.125,-87.125,Thompson Dock
diff --git a/data/csvCache/gnosis.json b/data/csvCache/gnosis.json
new file mode 100644
index 0000000..c843c8e
--- /dev/null
+++ b/data/csvCache/gnosis.json
@@ -0,0 +1,1218 @@
+[
+ {
+ "Route": "exploration",
+ "System": "Varati",
+ "x": "-178.65625",
+ "y": "77.125",
+ "z": "-87.125",
+ "Dates Visited": "May 18, 3303 - September 28, 3303"
+ },
+ {
+ "Route": "exploration",
+ "System": "Hyades Sector AQ-Y d81",
+ "x": "-83.4375",
+ "y": "-82.78125",
+ "z": "-244.21875",
+ "Dates Visited": "September 28, 3303 - October 5, 3303"
+ },
+ {
+ "Route": "exploration",
+ "System": "HIP 18778",
+ "x": "11.46875",
+ "y": "-236.5625",
+ "z": "-398.21875",
+ "Dates Visited": "October 5, 3303 - October 12, 3303"
+ },
+ {
+ "Route": "exploration",
+ "System": "Witch Head Sector DL-Y d8",
+ "x": "351.75",
+ "y": "-419.65625",
+ "z": "-706.5",
+ "Dates Visited": "October 12, 3303 - October 26, 3303"
+ },
+ {
+ "Route": "exploration",
+ "System": "BD-12 1172",
+ "x": "577.875",
+ "y": "-452.6875",
+ "z": "-819.25",
+ "Dates Visited": "October 26, 3303 - November 2, 3303"
+ },
+ {
+ "Route": "exploration",
+ "System": "Mintaka",
+ "x": "270.625",
+ "y": "-204.34375",
+ "z": "-603.78125",
+ "Dates Visited": "November 2, 3303 - November 9, 3303"
+ },
+ {
+ "Route": "exploration",
+ "System": "Betelgeuse",
+ "x": "169.40625",
+ "y": "-72.5",
+ "z": "-462.625",
+ "Dates Visited": "November 9, 3303 - November 16, 3303"
+ },
+ {
+ "Route": "exploration",
+ "System": "Merope",
+ "x": "-78.59375",
+ "y": "-149.625",
+ "z": "-340.53125",
+ "Dates Visited": "November 16, 3303 - November 23, 3303"
+ },
+ {
+ "Route": "exploration",
+ "System": "Meene",
+ "x": "118.78125",
+ "y": "-56.4375",
+ "z": "-97.1875",
+ "Dates Visited": "November 23, 3303 - December 7, 3303"
+ },
+ {
+ "Route": "exploration",
+ "System": "IC 2391 Sector YE-A d103",
+ "x": "489.03125",
+ "y": "-98.09375",
+ "z": "-34.96875",
+ "Dates Visited": "December 7, 3303 - December 14, 3303"
+ },
+ {
+ "Route": "exploration",
+ "System": "Jackson's Lighthouse",
+ "x": "157",
+ "y": "-27",
+ "z": "-70",
+ "Dates Visited": "December 14, 3303 - December 21, 3303"
+ },
+ {
+ "Route": "exploration",
+ "System": "Santa Muerte",
+ "x": "94.25",
+ "y": "46.1875",
+ "z": "58.03125",
+ "Dates Visited": "December 21, 3303 - December 28, 3303"
+ },
+ {
+ "Route": "exploration",
+ "System": "Varati",
+ "x": "-178.65625",
+ "y": "77.125",
+ "z": "-87.125",
+ "Dates Visited": "December 28, 3303 - January 11, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "LBN 623 Sector PD-S b4-5",
+ "x": "-499.90625",
+ "y": "-16.4375",
+ "z": "-332.5625",
+ "Dates Visited": "January 11, 3304 - January 18, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "HIP 17125",
+ "x": "-95.875",
+ "y": "-128.75",
+ "z": "-316.5625",
+ "Dates Visited": "January 18, 3304 - January 25, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Taygeta",
+ "x": "-88.5",
+ "y": "-159.6875",
+ "z": "-366.28125",
+ "Dates Visited": "January 25, 3304 - February 1, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Electra",
+ "x": "-86.0625",
+ "y": "-159.9375",
+ "z": "-361.65625",
+ "Dates Visited": "February 1, 3304 - Feburary 15, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Maia",
+ "x": "-81.78125",
+ "y": "-149.4375",
+ "z": "-343.375",
+ "Dates Visited": "Feburary 15, 3304 - March 8, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Evenses",
+ "x": "-133.875",
+ "y": "54.03125",
+ "z": "-80.5625",
+ "Dates Visited": "March 8, 3304 - March 15, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Meene",
+ "x": "118.78125",
+ "y": "-56.4375",
+ "z": "-97.1875",
+ "Dates Visited": "March 15, 3304 - March 22, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Synuefe XO-P c22-17",
+ "x": "546.90625",
+ "y": "-56.46875",
+ "z": "-97.8125",
+ "Dates Visited": "March 22, 3304 - March 29, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Vela Dark Region EG-X b1-1",
+ "x": "957.6875",
+ "y": "-143.34375",
+ "z": "-123.40625",
+ "Dates Visited": "March 29, 3304 - April 5, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "HD 63154",
+ "x": "979.46875",
+ "y": "-207.40625",
+ "z": "-131.59375",
+ "Dates Visited": "April 5, 3304 - April 12, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Vela Dark Region JS-T b3-0",
+ "x": "899.9375",
+ "y": "-142.1875",
+ "z": "-82.25",
+ "Dates Visited": "April 12, 3304 - April 19, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Synuefe EU-Q c21-10",
+ "x": "758.65625",
+ "y": "-176.90625",
+ "z": "-133.21875",
+ "Dates Visited": "April 19, 3304 - April 26, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Synuefe GT-H b43-1",
+ "x": "749",
+ "y": "-163.09375",
+ "z": "-128.0625",
+ "Dates Visited": "April 26, 3304 - May 3, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "HIP 39768",
+ "x": "866.59375",
+ "y": "-119.125",
+ "z": "-109.03125",
+ "Dates Visited": "May 3, 3304 - May 10, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Col 173 Sector ME-P d6-92",
+ "x": "891.65625",
+ "y": "-98.53125",
+ "z": "-157.1875",
+ "Dates Visited": "May 10, 3304 - May 17, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "IC 2391 Sector YE-A d103",
+ "x": "489.03125",
+ "y": "-98.09375",
+ "z": "-34.96875",
+ "Dates Visited": "May 17, 3304 - May 24, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Meene",
+ "x": "118.78125",
+ "y": "-56.4375",
+ "z": "-97.1875",
+ "Dates Visited": "May 24, 3304 - May 31, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Epsilon Indi",
+ "x": "3.125",
+ "y": "-8.875",
+ "z": "7.125",
+ "Dates Visited": "May 31, 3304 - June 21, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Jackson's Lighthouse",
+ "x": "157",
+ "y": "-27",
+ "z": "-70",
+ "Dates Visited": "June 21, 3304 - June 28, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Alpha Centauri",
+ "x": "3.03125",
+ "y": "-0.09375",
+ "z": "3.15625",
+ "Dates Visited": "June 28, 3304 - July 5, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "LHS 3447",
+ "x": "-43.1875",
+ "y": "-5.28125",
+ "z": "56.15625",
+ "Dates Visited": "July 5, 3304 - July 12, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Charick Drift",
+ "x": "8.1875",
+ "y": "26.71875",
+ "z": "-6.0625",
+ "Dates Visited": "July 12, 3304 - July 19, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Hermitage",
+ "x": "-28.75",
+ "y": "25",
+ "z": "10.4375",
+ "Dates Visited": "July 19, 3304 - July 26, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Bifrost",
+ "x": "58.6875",
+ "y": "54.28125",
+ "z": "-62.46875",
+ "Dates Visited": "July 26, 3304 - August 9, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Merope",
+ "x": "-78.59375",
+ "y": "-149.625",
+ "z": "-340.53125",
+ "Dates Visited": "August 9, 3304 - August 16, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "64 Orionis",
+ "x": "129.34375",
+ "y": "-7.1875",
+ "z": "-706.65625",
+ "Dates Visited": "August 16, 3304 - August 23, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "HD 51502",
+ "x": "411.25",
+ "y": "168.5625",
+ "z": "-1054.59375",
+ "Dates Visited": "August 23, 3304 - August 30, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Outotz ST-I d9-4",
+ "x": "609.4375",
+ "y": "154.25",
+ "z": "-1503.59375",
+ "Dates Visited": "August 30, 3304 - September 6, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Outotz ST-I d9-6",
+ "x": "604.15625",
+ "y": "153.59375",
+ "z": "-1514.9375",
+ "Dates Visited": "September 6, 3304 - September 27, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Outotz EH-M d7-5",
+ "x": "134.375",
+ "y": "208.40625",
+ "z": "-1664.6875",
+ "Dates Visited": "September 27, 3304 - October 4, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Outopps BU-R d4-4",
+ "x": "-284.78125",
+ "y": "235.1875",
+ "z": "-1906.84375",
+ "Dates Visited": "October 4, 3304 - October 11, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Outopps JB-X d1-1",
+ "x": "-705.53125",
+ "y": "251.15625",
+ "z": "-2145.90625",
+ "Dates Visited": "October 11, 3304 - October 18, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Ploi Thua YD-E c26-1",
+ "x": "-1101.25",
+ "y": "326.5625",
+ "z": "-2495.1875",
+ "Dates Visited": "October 18, 3304 - October 25, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Hegeia SY-O c20-0",
+ "x": "-1581.40625",
+ "y": "357.40625",
+ "z": "-2725.21875",
+ "Dates Visited": "October 25, 3304 - November 1, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Outorst WI-B d0",
+ "x": "-1822.09375",
+ "y": "545.9375",
+ "z": "-2338.96875",
+ "Dates Visited": "November 1, 3304 - November 8, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Outorst CW-K c9-0",
+ "x": "-2051.3125",
+ "y": "496.5625",
+ "z": "-1911.25",
+ "Dates Visited": "November 8, 3304 - November 15, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Outorst OA-U c17-0",
+ "x": "-2365.375",
+ "y": "310.28125",
+ "z": "-1579.40625",
+ "Dates Visited": "November 15, 3304 - November 22, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "NGC 7822 Sector BQ-Y d12",
+ "x": "-2454.1875",
+ "y": "299.0625",
+ "z": "-1326.0625",
+ "Dates Visited": "November 22, 3304 - November 29, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Plaa Eurk IR-W d1-14",
+ "x": "-2387.0625",
+ "y": "124",
+ "z": "-877.71875",
+ "Dates Visited": "November 29, 3304 - December 6, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Elephant's Trunk Sector BQ-Y d14",
+ "x": "-2670.84375",
+ "y": "224.46875",
+ "z": "-479.5625",
+ "Dates Visited": "December 6, 3304 - December 13, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Plaa Eurk QZ-D b26-0",
+ "x": "-2182.9375",
+ "y": "119.84375",
+ "z": "-500.25",
+ "Dates Visited": "December 13, 3304 - December 20, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Plaa Eurk JB-O d6-12",
+ "x": "-1687.53125",
+ "y": "146.5625",
+ "z": "-451.71875",
+ "Dates Visited": "December 20, 3304 - December 27, 3304"
+ },
+ {
+ "Route": "exploration",
+ "System": "Wredguia HO-Y c14-3",
+ "x": "-1189.46875",
+ "y": "145.46875",
+ "z": "-418.78125",
+ "Dates Visited": "December 27, 3304 - January 3, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Wredguia UE-V b30-1",
+ "x": "-691",
+ "y": "115.28125",
+ "z": "-397.84375",
+ "Dates Visited": "January 3, 3305 - January 10, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "MEL 22 Sector TB-J b10-0",
+ "x": "-245.21875",
+ "y": "-108.875",
+ "z": "-393.0625",
+ "Dates Visited": "January 10, 3305 - January 17, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "HR 1185",
+ "x": "-64.65625",
+ "y": "-148.9375",
+ "z": "-330.40625",
+ "Dates Visited": "January 17, 3305 - January 24, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Varati",
+ "x": "-178.65625",
+ "y": "77.125",
+ "z": "-87.125",
+ "Dates Visited": "January 24, 3305 - January 31, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Alpha Centauri",
+ "x": "3.03125",
+ "y": "-0.09375",
+ "z": "3.15625",
+ "Dates Visited": "January 31, 3305 - February 7, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Meene",
+ "x": "118.78125",
+ "y": "-56.4375",
+ "z": "-97.1875",
+ "Dates Visited": "February 7, 3305 - February 14, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "IC 2391 Sector HG-X b1-8",
+ "x": "603.9375",
+ "y": "-104.15625",
+ "z": "-61.5625",
+ "Dates Visited": "February 14, 3305 - February 21, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "HIP 36823",
+ "x": "640.4375",
+ "y": "-143.90625",
+ "z": "-118.3125",
+ "Dates Visited": "February 21, 3305 - February 28, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "HIP 39890",
+ "x": "648.5625",
+ "y": "-137.21875",
+ "z": "-0.3125",
+ "Dates Visited": "February 28, 3305 - March 7, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Synuefe LQ-T b50-1",
+ "x": "728.5",
+ "y": "-155.65625",
+ "z": "19.3125",
+ "Dates Visited": "March 7, 3305 - March 14, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Synuefe SP-F b44-0",
+ "x": "831.625",
+ "y": "-187.5625",
+ "z": "-121.84375",
+ "Dates Visited": "March 14, 3305 - March 21, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Col 173 Sector OD-J b25-2",
+ "x": "1008.375",
+ "y": "-201.5625",
+ "z": "-157.4375",
+ "Dates Visited": "March 21, 3305 - March 28, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Vela Dark Region FL-Y d63",
+ "x": "1064.5",
+ "y": "-144.03125",
+ "z": "-101.71875",
+ "Dates Visited": "March 28, 3305 - April 4, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Synuefe VN-W b46-0",
+ "x": "879.03125",
+ "y": "-604.78125",
+ "z": "-63.96875",
+ "Dates Visited": "April 4, 3305 - April 11, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Synuefe FE-S b46-0",
+ "x": "679.34375",
+ "y": "-1060.65625",
+ "z": "-62.25",
+ "Dates Visited": "April 11, 3305 - April 18, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Flyooe Dryeia LH-L c24-0",
+ "x": "456.34375",
+ "y": "-1503.59375",
+ "z": "-21.875",
+ "Dates Visited": "April 18, 3305 - April 25, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Flyooe Dryeia JJ-G d11-0",
+ "x": "228.21875",
+ "y": "-1936.625",
+ "z": "-67.875",
+ "Dates Visited": "April 25, 3305 - May 2, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "HIP 4099",
+ "x": "46.65625",
+ "y": "-2380.28125",
+ "z": "-9.15625",
+ "Dates Visited": "May 2, 3305 - May 9, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Prooe Dryeia XP-X d1-0",
+ "x": "20.15625",
+ "y": "-2099.9375",
+ "z": "383.8125",
+ "Dates Visited": "May 9, 3305 - May 16, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Prooe Dryeia SF-N d7-0",
+ "x": "-57.3125",
+ "y": "-2024.1875",
+ "z": "865.59375",
+ "Dates Visited": "May 16, 3305 - May 23, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Prooe Dryeia RQ-C d13-0",
+ "x": "61.59375",
+ "y": "-1980.5625",
+ "z": "1346.78125",
+ "Dates Visited": "May 23, 3305 - May 30 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Screagi FP-Z d0",
+ "x": "-115.8125",
+ "y": "-2001.625",
+ "z": "1588.40625",
+ "Dates Visited": "May 30, 3305 - June 6, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "IRAS 21565-3937",
+ "x": "-86.25",
+ "y": "-2376.875",
+ "z": "1828.375",
+ "Dates Visited": "June 6, 3305 - June 13, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Screagi BJ-B d0",
+ "x": "-138.3125",
+ "y": "-2015.75",
+ "z": "1501.21875",
+ "Dates Visited": "June 13, 3305 - June 20, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Nidgiae QJ-F d12-1",
+ "x": "-152.28125",
+ "y": "-1580.9375",
+ "z": "1274.65625",
+ "Dates Visited": "June 20, 3305 - June 27, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Swoilz II-K d8-0",
+ "x": "-53.9375",
+ "y": "-1217.09375",
+ "z": "949.53125",
+ "Dates Visited": "June 27, 3305 - July 4, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Swoilz GX-T d3-2",
+ "x": "-8.96875",
+ "y": "-851.65625",
+ "z": "613.65625",
+ "Dates Visited": "July 4, 3305 - July 11, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Swoilz UL-A c2-7",
+ "x": "18.5625",
+ "y": "-448.6875",
+ "z": "319.40625",
+ "Dates Visited": "July 11, 3305 - July 18, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Tonatiuh",
+ "x": "5.25",
+ "y": "-39.8125",
+ "z": "33.3125",
+ "Dates Visited": "July 18, 3305 - July 25, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "HIP 20960",
+ "x": "30.65625",
+ "y": "-189.53125",
+ "z": "-435",
+ "Dates Visited": "July 25, 3305 - August 1, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Witch Head Sector AL-X b1-0",
+ "x": "312.25",
+ "y": "-393.03125",
+ "z": "-773.46875",
+ "Dates Visited": "August 1, 3305 - August 8, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Wregoe JI-B b13-0",
+ "x": "44.6875",
+ "y": "-14.28125",
+ "z": "-782.9375",
+ "Dates Visited": "August 8, 3305 - August 15, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "California Sector LC-V c2-10",
+ "x": "-336.1875",
+ "y": "-237.78125",
+ "z": "-890.125",
+ "Dates Visited": "August 15, 3305 - August 22, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Oochorrs KF-D d13-5",
+ "x": "6.15625",
+ "y": "-469.3125",
+ "z": "-1150.28125",
+ "Dates Visited": "August 22, 3305 - August 29, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Col 69 Sector HR-M c7-6",
+ "x": "430.96875",
+ "y": "-427.15625",
+ "z": "-1331.75",
+ "Dates Visited": "August 29, 3305 - September 5, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Oort",
+ "x": "589.375",
+ "y": "-428.84375",
+ "z": "-1061.59375",
+ "Dates Visited": "September 5, 3305 - September 12, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Messier 78 Sector RD-T c3-8",
+ "x": "667.46875",
+ "y": "-426.96875",
+ "z": "-1314.125",
+ "Dates Visited": "September 12, 3305 - September 19, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Oochorrs FO-C c15-0",
+ "x": "643.71875",
+ "y": "-279.40625",
+ "z": "-1668",
+ "Dates Visited": "September 19, 3305 - September 26, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Outotz PI-K d8-0",
+ "x": "520.71875",
+ "y": "97.9375",
+ "z": "-1598.0625",
+ "Dates Visited": "September 26, 3305 - October 3, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Outotz ST-I d9-6",
+ "x": "604.15625",
+ "y": "153.59375",
+ "z": "-1514.9375",
+ "Dates Visited": "October 3, 3305 - October 10, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Col 69 Sector PH-U c3-0",
+ "x": "606.375",
+ "y": "-333.90625",
+ "z": "-1501.9375",
+ "Dates Visited": "October 10, 3305 - October 17, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "V1483 Orionis",
+ "x": "613.21875",
+ "y": "-446.25",
+ "z": "-1105",
+ "Dates Visited": "October 17, 3305 - October 24, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "PMD2009 48",
+ "x": "594.90625",
+ "y": "-431.4375",
+ "z": "-1071.78125",
+ "Dates Visited": "October 24, 3305 - October 31, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "BD-12 1172",
+ "x": "577.875",
+ "y": "-452.6875",
+ "z": "-819.25",
+ "Dates Visited": "October 31, 3305 - November 7, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Synuefe MJ-O d7-14",
+ "x": "489.78125",
+ "y": "-253.34375",
+ "z": "-378.25",
+ "Dates Visited": "November 7, 3305 - November 14, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Flame Sector XT-R b4-0",
+ "x": "497.40625",
+ "y": "-321.625",
+ "z": "-859.3125",
+ "Dates Visited": "November 14, 3305 - November 21, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "HIP 23759",
+ "x": "359.84375",
+ "y": "-385.53125",
+ "z": "-718.375",
+ "Dates Visited": "November 21, 3305 - November 28, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Synuefe NU-M d8-99",
+ "x": "370.96875",
+ "y": "-163.125",
+ "z": "-281.875",
+ "Dates Visited": "November 28, 3305 - December 5, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Epsilon Indi",
+ "x": "3.125",
+ "y": "-8.875",
+ "z": "7.125",
+ "Dates Visited": "December 5, 3305 - December 12, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "Varati",
+ "x": "-178.65625",
+ "y": "77.125",
+ "z": "-87.125",
+ "Dates Visited": "December 12, 3305 - December 26, 3305"
+ },
+ {
+ "Route": "exploration",
+ "System": "63 G. Capricorni",
+ "x": "-19.1875",
+ "y": "-44.8125",
+ "z": "52.53125",
+ "Dates Visited": "December 26, 3305 - January 2, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Alaunus",
+ "x": "-0.65625",
+ "y": "-52.28125",
+ "z": "-5.96875",
+ "Dates Visited": "January 2, 3306 - January 9, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "HIP 28170",
+ "x": "87.59375",
+ "y": "-18.40625",
+ "z": "-494.53125",
+ "Dates Visited": "January 9, 3306 - January 16, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Arietis Sector ZF-O b6-1",
+ "x": "-89.53125",
+ "y": "-102.4375",
+ "z": "-75.4375",
+ "Dates Visited": "January 16, 3306 - January 23, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "HIP 112240",
+ "x": "-132.59375",
+ "y": "-133.4375",
+ "z": "30.90625",
+ "Dates Visited": "January 23, 3306 - January 30, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Col 359 Sector TN-T d3-98",
+ "x": "-280.1875",
+ "y": "-146.1875",
+ "z": "445.6875",
+ "Dates Visited": "January 30, 3306 - February 6, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Col 359 Sector OI-K d8-10",
+ "x": "-530.21875",
+ "y": "-328.65625",
+ "z": "827.75",
+ "Dates Visited": "February 6, 3306 - February 13, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Swoiwns RJ-Q e5-2",
+ "x": "-708.21875",
+ "y": "-427.71875",
+ "z": "1232.625",
+ "Dates Visited": "February 13, 3306 - February 20, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Bleia Eohn TJ-Y d1-1",
+ "x": "-928.46875",
+ "y": "-469",
+ "z": "1661.9375",
+ "Dates Visited": "February 20, 3306 - February 27, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Bleia Eohn PE-P d6-5",
+ "x": "-1091.5",
+ "y": "-622.5625",
+ "z": "2102.28125",
+ "Dates Visited": "February 27, 3306 - March 5, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Bleia Eohn UO-K c23-0",
+ "x": "-1326.40625",
+ "y": "-718.75",
+ "z": "2524.21875",
+ "Dates Visited": "March 5, 3306 - March 12, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Drojeae WZ-M b9-0",
+ "x": "-1504.84375",
+ "y": "-821.4375",
+ "z": "2978.09375",
+ "Dates Visited": "March 12, 3306 - March 19, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Drojeae NM-M d7-0",
+ "x": "-1580.84375",
+ "y": "-994.25",
+ "z": "3428.84375",
+ "Dates Visited": "March 19, 3306 - March 26, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Drojeae UE-H d10-0",
+ "x": "-1912.1875",
+ "y": "-986.9375",
+ "z": "3724.8125",
+ "Dates Visited": "March 26, 3306 - April 2, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Blae Drye FF-A d2",
+ "x": "-2009.6875",
+ "y": "-1215.09375",
+ "z": "4114.375",
+ "Dates Visited": "April 2, 3306 - April 9, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Blae Drye PS-U e2-0",
+ "x": "-2137.28125",
+ "y": "-1287.96875",
+ "z": "4555.75",
+ "Dates Visited": "April 9, 3306 - April 16, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Blae Dryua RL-J d10-2",
+ "x": "-2371.84375",
+ "y": "-1332.90625",
+ "z": "4966.875",
+ "Dates Visited": "April 16, 3306 - April 23, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Chaluia FS-A d1-4",
+ "x": "-2486.15625",
+ "y": "-1483.625",
+ "z": "5426.65625",
+ "Dates Visited": "April 23, 3306 - April 30, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Blae Drye YK-P e5-1",
+ "x": "-2497.1875",
+ "y": "-1274.75",
+ "z": "5058.8125",
+ "Dates Visited": "April 30, 3306 - May 7, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Blae Drye CH-M D7-4",
+ "x": "-2597.9375",
+ "y": "-1078.90625",
+ "z": "4711.1875",
+ "Dates Visited": "May 7, 3306 - May 14, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Blae Drye GY-P c6-0",
+ "x": "-2542.75",
+ "y": "-819.4375",
+ "z": "4335.375",
+ "Dates Visited": "May 14, 3306 - May 21, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Drojo TO-E c26-2",
+ "x": "-2655.21875",
+ "y": "-894.75",
+ "z": "3899.15625",
+ "Dates Visited": "May 21, 3306 - May 28, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Drojo DP-X c15-1",
+ "x": "-2742",
+ "y": "-982.5625",
+ "z": "3456.3125",
+ "Dates Visited": "May 28, 3306 - June 4, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Drojo FA-Q d5-6",
+ "x": "-2821.53125",
+ "y": "-1020.625",
+ "z": "3288.90625",
+ "Dates Visited": "June 4, 3306 - June 11, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Drojeae DB-X D1-4",
+ "x": "-2504.0625",
+ "y": "-1057.65625",
+ "z": "2968",
+ "Dates Visited": "June 11, 3306 - June 18, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Drojeae AA-A h56",
+ "x": "-2164.59375",
+ "y": "-733.40625",
+ "z": "2923.875",
+ "Dates Visited": "June 18, 3306 - June 25, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Aucopp QO-U b47-0",
+ "x": "-1885.34375",
+ "y": "-624.21875",
+ "z": "2524.03125",
+ "Dates Visited": "June 25, 3306 - July 2, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Aucopp VJ-P d6-2",
+ "x": "-1670.5625",
+ "y": "-581.96875",
+ "z": "2094.21875",
+ "Dates Visited": "July 2, 3306 - July 9, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Aucopp BP-Y d1-35",
+ "x": "-1407.875",
+ "y": "-422.0625",
+ "z": "1704.3125",
+ "Dates Visited": "July 9, 3306 - July 16, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Phylur AP-B b13-1",
+ "x": "-1617.90625",
+ "y": "-1.65625",
+ "z": "1775.4375",
+ "Dates Visited": "July 16, 3306 - July 23, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Sifi PC-V b57-0",
+ "x": "-1999.53125",
+ "y": "20.21875",
+ "z": "1455.46875",
+ "Dates Visited": "July 23, 3306 - July 30, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Sifi BA-E B39-0",
+ "x": "-1799.65625",
+ "y": "-21.5",
+ "z": "1057.8125",
+ "Dates Visited": "July 30, 3306 - August 6, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Star of India",
+ "x": "-1840.5625",
+ "y": "95.25",
+ "z": "610.3125",
+ "Dates Visited": "August 6, 3306 - August 13, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Veil West Sector AQ-Y d43",
+ "x": "-1448.875",
+ "y": "-157.96875",
+ "z": "444.1875",
+ "Dates Visited": "August 13, 3306 - August 20, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Dumbbell Sector CL-X b1-2",
+ "x": "-979.5625",
+ "y": "-73.90625",
+ "z": "484.96875",
+ "Dates Visited": "August 20, 3306 - August 27, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Col 359 Sector MJ-D b14-3",
+ "x": "-603.875",
+ "y": "2.6875",
+ "z": "422.5625",
+ "Dates Visited": "August 27, 3306 - September 3, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "HIP 98182",
+ "x": "-545.90625",
+ "y": "7.09375",
+ "z": "223.1875",
+ "Dates Visited": "September 3, 3306 - September 10, 3306"
+ },
+ {
+ "Route": "exploration",
+ "System": "Varati",
+ "x": "-178.65625",
+ "y": "77.125",
+ "z": "-87.125",
+ "Dates Visited": "September 10, 3306 - September 17, 3306"
+ },
+ {
+ "Route": "final",
+ "System": "Varati",
+ "x": "-178.65625",
+ "y": "77.125",
+ "z": "-87.125",
+ "Dates Visited": ""
+ },
+ {
+ "Route": "final",
+ "System": "HIP 17862",
+ "x": "-81.4375",
+ "y": "-151.90625",
+ "z": "-359.59375",
+ "Dates Visited": ""
+ },
+ {
+ "Route": "final",
+ "System": "Pleiades Sector PN-T b3-0",
+ "x": "-79.53125",
+ "y": "-199.9375",
+ "z": "-361.59375",
+ "Dates Visited": ""
+ },
+ {
+ "Route": "final",
+ "System": "Synuefe PR-L b40-1",
+ "x": "365.78125",
+ "y": "-291.75",
+ "z": "-188.65625",
+ "Dates Visited": ""
+ },
+ {
+ "Route": "final",
+ "System": "HIP 18120",
+ "x": "345.75",
+ "y": "-435.71875",
+ "z": "-125.5",
+ "Dates Visited": ""
+ },
+ {
+ "Route": "final",
+ "System": "IC 2391 Sector CQ-Y c16",
+ "x": "559.875",
+ "y": "-87.15625",
+ "z": "-33.15625",
+ "Dates Visited": ""
+ },
+ {
+ "Route": "final",
+ "System": "Kappa-1 Volantis",
+ "x": "396.90625",
+ "y": "-142.5625",
+ "z": "106.09375",
+ "Dates Visited": ""
+ },
+ {
+ "Route": "final",
+ "System": "Epsilon Indi",
+ "x": "3.125",
+ "y": "-8.875",
+ "z": "7.125",
+ "Dates Visited": ""
+ }
+]
\ No newline at end of file
diff --git a/data/csvCache/hesperus.csv b/data/csvCache/hesperus.csv
new file mode 100644
index 0000000..ba6ecd7
--- /dev/null
+++ b/data/csvCache/hesperus.csv
@@ -0,0 +1,29 @@
+name,pos_x,pos_y,pos_z,category,infos
+PMD2009 48,594.90625 , -431.4375 , -1071.78125,,Orion Nebula Tourist Centre
+Trapezium Sector AF-Z c0, 617.03125 , -421.625 , -1224.5625,,Barnard's Loop
+Merope,-78.59375 , -149.625 , -340.53125,,
+Sol,0,0,0,,
+Col 70 Sector FY-N c21-3, 687.0625 , -362.53125 , -697.0625,,Thargoid Probe
+Perseus Dark Region KC-V C2-2,-368.03125 , -321.6875 , -1047.625,201,The Hesperus a Lowell class megaship owned by Azimuth Biochemicals. It is the sister ship of the Adamastor alongside a dredger from the Scriveners Clan which is claiming to own to salvage rights on the Hesperus.
+Taurus Dark Region UY-R B4-0, -3.59375 , -120.4375 , -444.5625,203,Listening Post with the nickname "H1" found inside an Asteroid Belt through the Listening Post inside Adamastor (Chukchan).
+Perseus Dark Region LN-K B8-0, -363.78125 , -380.78125 , -974.53125,203,Listening Post with the nickname "H2" found inside an Asteroid Belt through decyphering of H1.
+California Sector IH-V C2-1,-352.03125 , -222.65625 , -885.21875,203,Listening Post with the nickname "H3" found through decyphering of H1.
+Li Chul, 120.03125 , 60.21875 , 44.71875,201,According to Salvation: Li Chul is the Hesperus starting system - Hesperus 17 Listening Post Challenge begins here.
+HIP 44101,156.6875 , 22.28125 , -33.0625,202,Beacon #1: AZB2/H002255/TX-B-01
found by CMDR Fundevin
+Col 285 Sector WH-O a22-2,143.75 , -33.5625 , -106.53125,202,Beacon #2: AZB2/H002255/TX-B-02
found by CMDR sawley
+COL 285 Sector NV-N B7-4,168.0625 , -73.90625 , -192,202,Beacon #3: AZB2/H002255/TX-B-03
found by CMDR Namix
+Synuefe FM-S B37-4,208.71875 , -138.84375 , -255.4375,202,Beacon #4: AZB2/H002255/TX-B-04
found by CMDR itsbeanmachine
+Synuefe BA-X B34-2,198.65625 , -214.125 , -309.8125,202,Beacon #5: AZB2/H002255/TX-B-05
found by CMDR Mong Morg
+Synuefe JJ-O d7-55,191.5625 , -259.1875 , -391.71875,202,Beacon #6: AZB2/H002255/TX-B-06
found by CMDR Balvald
+Synuefe HY-P D6-31,215.96875 , -306.125 , -468.40625,202,Beacon #7: AZB2/H002255/TX-B-07
it is not known who found this one
+Synuefe MQ-H C12-4,268.21875 , -328.25 , -544.3125,202,Beacon #8: AZB2/H002255/TX-B-08
found by CMDR itsbeanmachine
+Synuefe RJ-U B21-0,330.46875 , -357.65625 , -603.4375,202,Beacon #9: AZB2/H002255/TX-B-09
found by CMDR WesUAH / CMDR Lurch The Bastard
+Witch head sector OT-Q B5-0, 337.625 , -359.71875 , -701.125,202,Beacon #10: AZB2/H002255/TX-B-10
found by CMDR The Shrike
+Horsehead Sector CB-O b6-0,376.8125 , -332.875 , -784.84375,202,Beacon #11: AZB2/H002255/TX-B-11
found by CMDR snedex
+Flame Sector PI-T b3-0,378.59375 , -340.6875 , -882.15625,202,Beacon #12: AZB2/H002255/TX-B-12
found by CMDR bagofholding
+NGC 1999 Sector HN-S B4-0,455.625 , -343.8125 , -941.4375,202,Beacon #13: AZB2/H002255/TX-B-13
found by CMDR DaftMav
+NGC 1999 Sector ZU-X b1-0,536.375 , -339.3125 , -991.59375,202,Beacon #14: AZB2/H002255/TX-B-14
found by CMDR Trident411
+Trapezium Sector FH-U c3-3, 507.25 , -336.1875 , -1078.125,202,Beacon #15: AZB2/H002255/TX-B-15
found by CMDR Trident411
+Col 69 Sector VK-E c12-10,440.84375 , -309.40625 , -1136.9375,202,Beacon #16: AZB2/H002255/TX-B-16
found by CMDR Sir_Benton
+Col 69 sector LY-H C10-0,359.75 , -325.03125 , -1185.3125,202,Beacon #17: AZB2/H002255/TX-B-17
found by CMDR Zador
+Trapezium sector YU-X C1-2, 573.59375 , -339.46875 , -1167.65625,100,The Proteus - Survivors of the Hesperus of Azimuth Biochemicals
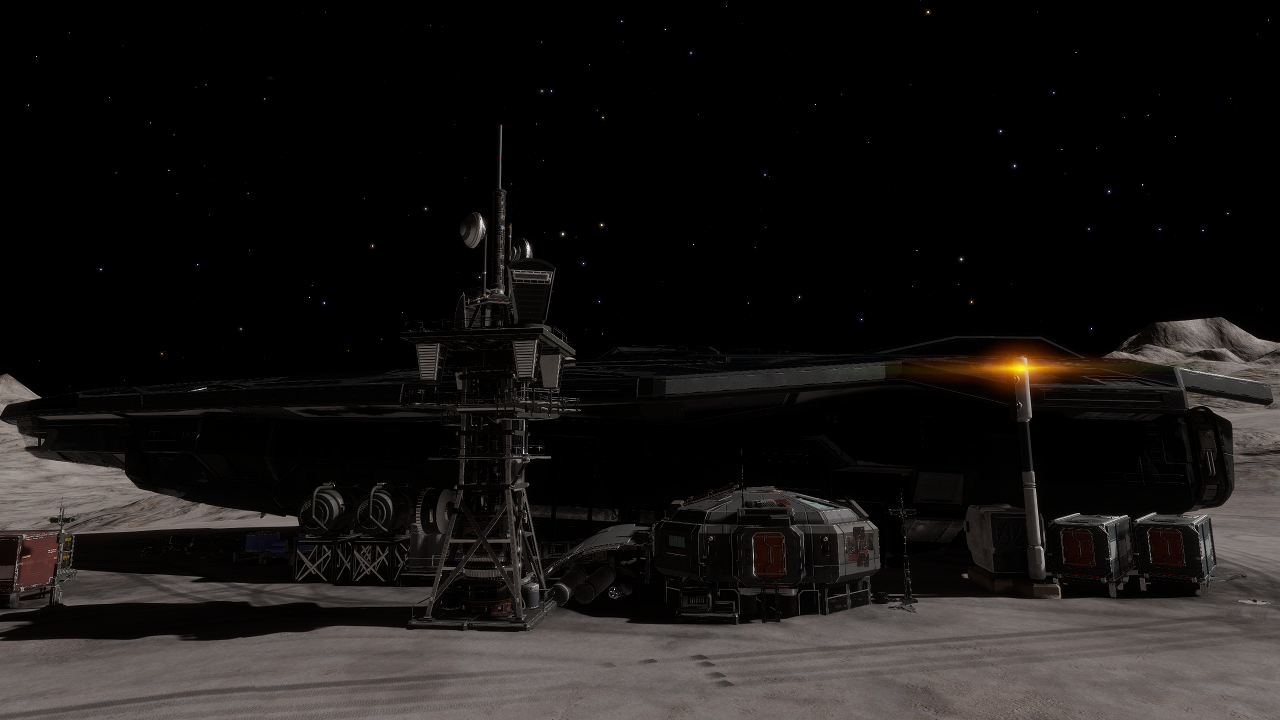
The Proteus is an Anaconda on planet 1 a. It can be found in a Point of Interest named AZB2/H002255/SC-01.
\ No newline at end of file
diff --git a/data/csvCache/notable_systems.json b/data/csvCache/notable_systems.json
new file mode 100644
index 0000000..f6e4f11
--- /dev/null
+++ b/data/csvCache/notable_systems.json
@@ -0,0 +1,157 @@
+[
+ {
+ "category": "Guardian",
+ "entry_name": "Hen 2-333",
+ "system": "Hen 2-333",
+ "x": "-840.65625",
+ "y": "-561.15625",
+ "z": "13361.8125",
+ "html": "This is at the centre of a permit very similar to the Regor Sector Permit, and is theorised to be of significance to the Guardians
The Aronnax Expedition reached the vicinity of the permit-locked IC 4673 Sector on 19th January 3304 and spent one week surveying the borders for signs of spacefaring activity, as well as outdoor worlds and systems with all the materials needed for FSD boosting ('green' systems). The region was also circumnavigated to establish its size and morphology, which revealed it to be a sphere of 100 LY radius centred on the Wolf-Rayet system Hen 2-333, which is a possible supernova candidate and is surrounded by a tiny nebula consisting of mass thrown off the central star.
It is currently unknown whether the permit lock is a result of a safety precaution or interference from astrophysical phenomena, such as extreme radiation resulting from a supernova, however the immediate vicinity of the border was discovered to have an unusual abundance of life-bearing worlds, including two systems that each harbour two Earth-like Worlds, that must all be considered endangered.
"
+ },
+ {
+ "category": "Guardian",
+ "entry_name": "Gamma Velorum",
+ "system": "Gamma Velorum",
+ "x": "1099.21875",
+ "y": "-146.6875",
+ "z": "-133.59375",
+ "html": "Gamma Velorum is a multiple star system in the constellation Vela. At magnitude +1.7, it is one of the brightest stars in the night sky, and by far the closest and brightest Wolf-Rayet star. It has the traditional names Suhail and Suhail al Muhlif, which confusingly also apply to Lambda Velorum. It also has a more modern popular name Regor.
This is one of the main theorised locations of the Guardian Homeworld, due to it being the centre of the main guardian bubble and being a hotter than avg star, such as where the guardians first evolved
"
+ },
+ {
+ "category": "Human",
+ "entry_name": "Sol",
+ "system": "Sol",
+ "x": "0",
+ "y": "0",
+ "z": "0",
+ "html": "The birthplace of humanity and the capital system of the Federation.
The galactic coordinate system is defined with Sol as the center (coordinates 0.0.0).
The Federation is the oldest of the ‘Big Three’ human superpowers. It has been in existence for over a thousand years.
By 3300 the Federation remains the largest power, holding sway over dozens of star systems within the ‘bubble’ of space known as the core worlds. It maintains a significant military force in its navy, the pinnacle of which is the ‘Farragut’ Class battlecruiser, a vessel some 2 kilometres long. A deployment of this vessel tends to quickly end anything other than a very major military engagement.
The Federation remains driven by the corporations. Some of the most famous of them have histories entwined with the Federation. The Sirius corporation, perhaps the biggest of them all, operating out of the Sirius system, has a virtual monopoly on power generators and hyperdrive technology. Other manufacturers are famed throughout space; with names such as Core Dynamics, Lakon Spaceways, Whatt and Pritney, Durn and Resner, Faulcon DeLacy, Zorgon Peterson and Saud Kruger. These so called ‘mega-corporations’ control the birth, lives and deaths of their employees, providing for their every need and expecting absolute loyalty in return.
Technology is a major part of any federation citizen’s life, and the economy is driven by quite conspicuous (and often compulsory) consumption of new and exciting mod cons, luxuries and consumables. The society is very much ‘throwaway’ with the new and exciting replacing the ‘old and outdated’ often within months of acquisition. This drives a constant demand and supply culture, with employees spending the money they earn, further driving the success of the corporations. Strictly speaking the Federation is composed of ‘States’, in a similar manner to the U.S.A. of 21st century Earth, but on a much bigger scale. States can still be countries, but they could be entire systems. The Federation retains a presidential electoral system, but corporations influence this dramatically, expecting their employees to vote according to the corporation’s wishes. Corruption, bribery and underhanded influence are rife. Individual freedoms are suppressed in favour of profit. Greed is good.
The Federation’s capitalist model retains the economic advantages and disadvantages of its predecessors. There are many rich people, but there are many people in grinding poverty too, in such debt to the corporations that they can be regarded as wage-slaves.
"
+ },
+ {
+ "category": "Thargoid",
+ "entry_name": "Merope",
+ "system": "Merope",
+ "x": "-78.59375",
+ "y": "-149.625",
+ "z": "-340.53125",
+ "html": "
Starting in March 3301, independent pilots began to encounter Unknown Artifacts in space. These appeared to be of an alien and almost organic origin and this raised the interest of the galactic scientific community. Research revealed that these unknown artifacts where pointing towards the system of Merope, and that they where in fact to be found in a shell with a radius of approximately 150 LY around this system. This is also the approximate area of space where unknown alien ships has appeared to pull pilots out of hyperspace.
A closer investigation revealed barnacle sites to be present on Merope 2 A and 5 C, but apart from these nothing outstanding has been found in the system itself. At least, not for the time being...
Interest in Merope was renewed with the discovery of a network of Unknown Structures that also appears to form a shell-like structure around Merope. This interest has only increased with the revelation that both the unknown artifacts, ships, and structures may all be of Thargoid origin. But to this day it is still a mystery how Merope itself fits into the bigger picture.
"
+ },
+ {
+ "category": "Thargoid",
+ "entry_name": "Col 70 Sector FY-N c21-3",
+ "system": "Col 70 Sector FY-N c21-3",
+ "x": "687.06",
+ "y": "-362.53",
+ "z": "-697.06",
+ "html": "
Col 70 Sector FY-N c21-3 is one of two systems of great significance to the Thargoids. In the permit locked Col 70 sector, it cannot be visited but we know it is significant because it is used as a reference site for the Thargoid measure of interstellar distances. The thargoids measure distance relative to the distance between Merope and Col 70 Sector FY-N c21-3
"
+ },
+ {
+ "category": "INRA",
+ "entry_name": "Almeida Landing",
+ "system": "Conn",
+ "x": "-36.59375",
+ "y": "-9.1875",
+ "z": "-20.5",
+ "img": "https://cdn-5f3c0a05c1ac180394b62f3c.closte.com/wp-content/uploads/2017/11/Screenshot_2646.jpg",
+ "html": "Ameida Landing is an abandoned settlement on Conn A 3 a at 73.3853, 102.3709.
The location of this facility was given by an Unregistered Comms Beacon that can be found in orbit of Teegarden’s Star 2. Known to transmit the following at 45 minutes past every hour:
SECURE 9 14 18 1 RISKY MOVE DRIVE TECH WHAT CHOICE IGNORING EVERYTHING CONN A 3 A SECURE
(repeated four times)
The numerical sequence decodes by letter positions to INRA.
The facility was operated by the Intergalactic Naval Reserve Arm (INRA), a co-operative between the Federation and Empire that operated during the last war with the Thargoids. This facility appears to have been used to reverse-engineer Thargoid drive technology.
"
+ },
+ {
+ "category": "INRA",
+ "entry_name": "Mayes Chemical Plant",
+ "system": "HIP 59382",
+ "x": "19.03125",
+ "y": "130.09375",
+ "z": "-11.59375",
+ "img": "https://cdn-5f3c0a05c1ac180394b62f3c.closte.com/wp-content/uploads/2017/10/Screenshot_2547.jpg",
+ "html": "Mayes Chemical Plant is an abandoned settlement on HIP 59382 1 b at 11.3987, 177.0659.
The location of this facility was given in the logs found at Klatt Enterprises.
It was operated by the Intergalactic Naval Reserve Arm (INRA), a co-operative between the Federation and Empire that operated during the last war with the Thargoids. This facility appears to have had connections to the project that created the mycoid used against the Thargoids, operating as a production facility.
"
+ },
+ {
+ "category": "INRA",
+ "entry_name": "Hogan Depot",
+ "system": "HIP 7158",
+ "x": "-3.78125",
+ "y": "-143.84375",
+ "z": "-33.8125",
+ "img": "https://cdn-5f3c0a05c1ac180394b62f3c.closte.com/wp-content/uploads/2017/10/Screenshot_2555.jpg",
+ "html": "Hogan Depot is an abandoned settlement on HIP 7158 A 2 b at -44.6309, -63.7982.
The location of this facility was given in the logs found at Mayes Chemical Plant.
It was operated by the Intergalactic Naval Reserve Arm (INRA), a co-operative between the Federation and Empire that operated during the last war with the Thargoids. This facility appears to have had connections to the project that created the mycoid used against the Thargoids, operating as a transfer facility.
"
+ },
+ {
+ "category": "INRA",
+ "entry_name": "Velasquez Medical Research Center",
+ "system": "LP 389-95",
+ "x": "98.84375",
+ "y": "7",
+ "z": "-7.71875",
+ "img": "https://cdn-5f3c0a05c1ac180394b62f3c.closte.com/wp-content/uploads/2017/10/Screenshot_2556.jpg",
+ "html": "Velasquez Medical Research Centre is an abandoned settlement on LP 389-95 7 at 57.9668, 50.0331.
The location of this facility was given in the logs found at Hogan Depot.
It was operated by the Intergalactic Naval Reserve Arm (INRA), a co-operative between the Federation and Empire that operated during the last war with the Thargoids. This facility appears to have had connections to the project that created the mycoid used against the Thargoids, operating as a medical research laboratory.
The vaccine for the mycoid agent was developed here.
"
+ },
+ {
+ "category": "INRA",
+ "entry_name": "Hollis Gateway",
+ "system": "Hermitage",
+ "x": "-28.75",
+ "y": "25",
+ "z": "10.4375",
+ "img": "https://cdn-5f3c0a05c1ac180394b62f3c.closte.com/wp-content/uploads/2017/10/Screenshot_2608.jpg",
+ "html": "Hollis Gateway is an abandoned settlement on Hermitage 4 a at -53.7548, 157.5843.
The location of this facility was given in the logs found at Stuart Retreat, and at Klatt Enterprises.
It was operated by the Intergalactic Naval Reserve Arm (INRA), a co-operative between the Federation and Empire that operated during the last war with the Thargoids. This facility appears to have had connections to the project that created the mycoid used against the Thargoids, operating as an agricultural laboratory.
"
+ },
+ {
+ "category": "INRA",
+ "entry_name": "Taylor Keep",
+ "system": "12 Trianguli",
+ "x": "-77.15625",
+ "y": "-76.81250",
+ "z": "-120.68750",
+ "img": "https://cdn-5f3c0a05c1ac180394b62f3c.closte.com/wp-content/uploads/2017/12/Screenshot_0053.png",
+ "html": "
Taylor Keep is an abandoned settlement on 12 Trianguli A 1 at -51.5711, 130.6677.
\r\nThe location of this facility was given by an Unregistered Comms Beacon at Ain 2. The comms beacon transmits a message in phonetic alphabet.
\r\nTaylor Keep was operated by the Intergalactic Naval Reserve Arm (INRA), a co-operative between the Federation and Empire that operated during the last war with the Thargoids. This facility appears to have had connections to the project that created the mycoid used against the Thargoids, operating as a launch point for the delivery of weaponised mycoid to a Thargoid hive ship by Commander Jameson.
\r\nThe facility contains a number of habitation modules, hangar or barracks buildings, it is surrounded by defence turrets. A major feature is the ship landing pad and control tower. There are collectable materials scattered around the site.
\r\nWithin the facility there are four Data Points which may be scanned for an Intel Package, and for Engineer Data. There is a time limit to scanning the Data Points. The Data Point also will give you the waypoint for the site in the Navigation Panel.
\r\nAround the facility there are three Settlement Comms Log Uplink points, scanning them gives some of the history of the INRA experiments:
All Our Hopes 1/3
\r\n\u2026Bypassing security protocols\u2026
\r\n\u2026Secure connection established\u2026
\r\n\u2026Communications record 1/3\u2026
\r\nI\u2019ll admit I had my reservations about this Commander Jameson. I looked into his background and apparently he climbed the ranks of the Pilots Federation with unprecedented speed. He\u2019s revered by other pilots. But that doesn\u2019t mean he\u2019s suited to the task.
\r\nBut then it struck me \u2013 all we need is someone who can get the payload where it needs to go. The hard work \u2013 the development of the mycoid \u2013 has already been done. All Jameson has to do is push a button.
\r\nThe important thing is to play up the whole \u2018saving humanity\u2019 angle. Make him feel like a hero. That should stop him asking too many questions.
\r\nAnd if everything goes wrong, we can just pin the blame on him.\r\n
\r\nAll Our Hopes 2/3
\r\n\u2026Bypassing security protocols\u2026
\r\n\u2026Secure connection established\u2026
\r\n\u2026Communications record 2/3\u2026
\r\nHaving received the payload from the Alnath system we\u2019ve prepared a number of medium-range missiles loaded with the weaponised version of the mycoid.
\r\nJameson will be here in three days to prep for the mission, which should give us more than enough time to fit his vessel. Apparently he insisted on using his own ship. I\u2019ve also ordered that some special modifications be made to his craft.
\r\nHe\u2019ll have to get close to launch the missiles, so it\u2019s unlikely he\u2019ll be coming back. But if he does survive, there are contingencies in place.\r\n
\r\n\r\nAll Our Hopes 3/3
\r\n\u2026Bypassing security protocols\u2026
\r\n\u2026Secure connection established\u2026
\r\n\u2026Communications record 3/3\u2026
\r\nTo: Commander Jameson
\r\nFrom: Amaro Hem, Programme Coordinator
\r\nWelcome, Commander Jameson. Your reputation precedes you. We are extremely grateful you chose to accept this mission.
\r\nI understand that the purpose of the assignment has been explained to you, but allow me to reiterate: this mission, if executed successfully, could mean the end of our war with the Thargoids. Its importance cannot be overstated.
\r\nThe consignment has been loaded onto your ship. We have made some minor modification to your vessel, but it should not affect the performance or functionality in any way.
\r\nThis is an extremely dangerous mission, Commander. You will be heading deep into enemy territory. If anything goes wrong, you\u2019re on your own.
\r\nGood luck, Commander. The future of the human race is in your hands. All our hopes and prayers go with you.\r\n
"
+ },
+ {
+ "category": "INRA",
+ "entry_name": "Klatt Enterprises",
+ "system": "Alnath",
+ "x": "-3.87500",
+ "y": "-7.37500",
+ "z": "-133.65625",
+ "img": "https://cdn-5f3c0a05c1ac180394b62f3c.closte.com/wp-content/uploads/2017/10/Screenshot_2533.jpg",
+ "html": "Carmichael Point is an abandoned settlement on HIP 16824 A 2 f at 73.8762, 61.8761.
\r\nThe location of this facility was given by an Unregistered Comms Beacon at HR 1188 A 2. The comms beacon transmits a message in phonetic alphabet on the hour.
\r\nSecure 9 14 18 1 Bait Deployed Contact Confirmed HIP 16824 A 2 F Secure
\r\n9 14 18 1 are the letter offsets for INRA
\r\nCarmichael Point was operated by the Intergalactic Naval Reserve Arm (INRA), a co-operative between the Federation and Empire that operated during the last war with the Thargoids. This facility appears to have had connections to the project that created the mycoid used against the Thargoids, it was used to lure a Thargoid ship in order to attack it. The attack failed, so happened presumably before the attack made by Commander Jameson.
\r\nThe facility contains a number of habitation modules, hangar or barracks buildings, and has two large mycoid storage tanks. There are collectable materials scattered around the site.
\r\nWithin the facility there is a Data Point which may be scanned for an Intel Package, and for Engineer Data. The Data Point also will give you the waypoint for the site in the Navigation Panel.
\r\nAround the facility there are four Settlement Comms Log Uplink points, scanning them gives some of the history of the INRA experiments:
\r\n\r\nWatching The Sky 1/4
\r\n\u2026Bypassing security protocols\u2026
\r\n\u2026Secure connection established\u2026
\r\n\u2026Site records found\u2026
\r\nEverything is in place.
\r\nIf the thargoids take the bait, this facility should come under attack very soon. And when it does, we\u2019ll find out if our new weapons are worth a damn.
\r\nIt\u2019s taken a lot of time and effort to make this place look like an important military site. I just hope they fall for it. It\u2019s about time we started fighting back.\r\n
\r\n\r\nWatching The Sky 2/4
\r\n\u2026Bypassing security protocols\u2026
\r\n\u2026Secure connection established\u2026
\r\n\u2026Site records found\u2026
\r\nEveryone\u2019s on edge. It\u2019s like seeing an approaching storm and waiting for it to break. The air is heavy with the threat of violence.
\r\nMaybe they didn\u2019t take the bait? Maybe we hid it too well? Or maybe we didn\u2019t hide it well enough. What if they realise this is a trap?
\r\nI suppose it\u2019s too late to worry about that now. All we can do is watch the sky, and wait.\r\n
\r\n\r\nWatching The Sky 3/4
\r\n\u2026Bypassing security protocols\u2026
\r\n\u2026Secure connection established\u2026
\r\n\u2026Site records found\u2026
\r\nContact confirmed! Thargoids 1,000 light seconds from the site and closing. Ready all weapon systems and prepare to fire on my order. We\u2019ve only got one shot at this and I don\u2019t want to miss.
\r\nWait\u2026 wait. What is that? That\u2019s not a regular Thargoid ship. It\u2019s huge.
\r\nWill someone scan that thing! And tell command we\u2019ve got a mothership here. Get them the data as soon as possible.
\r\nAll right, all right, that\u2019s close enough. Fire all batteries!\r\n
\r\n\r\nWatching The Sky 4/4
\r\n\u2026Bypassing security protocols\u2026
\r\n\u2026Secure connection established\u2026
\r\n\u2026Site records found\u2026
\r\nTest unsuccessful\u2026targets suffered minimal damage\u2026site lost\u2026all operatives\u2026lost\u2026\r\n
\r\nThe INRA base found after Carmichael was Stack.
"
+ },
+ {
+ "category": "INRA",
+ "entry_name": "Stack",
+ "system": "HIP 12099",
+ "x": "-101.90625",
+ "y": "-95.46875",
+ "z": "-165.59375",
+ "img": "https://cdn-5f3c0a05c1ac180394b62f3c.closte.com/wp-content/uploads/2017/12/Screenshot_0051.png",
+ "html": "Stack is an abandoned settlement on HIP 12099 1 a at -72.6561, -67.3629.
\r\nThe location of this facility was given by an Unregistered Comms Beacon in HIP 8887. The comms beacon transmits a message in phonetic alphabet:
\r\n\r\nSECURE 9 1 4 1 8 1 HIP 7158 DELIVERY RECEIVED HIP 12099 1A SECURE.\r\n
\r\nThe \u20189 1 4 1 8 1\u2019 can be clustered as \u20189 14 18 1\u2019, which are the letter offsets for INRA. HIP 12099 1 a is a reference to Taylor Keep, HIP 7158 refers to Hogan Depot.
\r\nStack was operated by the Intergalactic Naval Reserve Arm (INRA), a co-operative between the Federation and Empire that operated during the last war with the Thargoids. This facility appears to have had connections to the project that created the mycoid used against the Thargoids, operating as a thargoid live experimentation laboratory.
\r\nThe facility contains a number of habitation modules, hangar or barracks buildings, and large tanks marked with the symbol for the mycoid weapon; the ground is stained in a manner consistent with leakage of the agent. A major feature is the experimentation pit (reached through a raised roadway) which seems to contains parts of Thargoids that have been experimented upon. There are collectable materials scattered around the site.
\r\nWithin the facility there is a Data Point which may be scanned for an Intel Package, and for Engineer Data. The Data Point also will give you the waypoint for the site in the Navigation Panel.
\r\nAround the facility there are four Settlement Comms Log Uplink points, scanning them gives some of the history of the INRA experiments:
\r\n"
+ },
+ {
+ "category": "INRA",
+ "entry_name": "Stuart Retreat",
+ "system": "HIP 15329",
+ "x": "-87.84375",
+ "y": "-52.00000",
+ "z": "-167.37500",
+ "img": "https://cdn-5f3c0a05c1ac180394b62f3c.closte.com/wp-content/uploads/2017/10/Screenshot_2563.jpg",
+ "html": "
Stuart Retreat is an abandoned settlement on HIP 15329 A 3 c at -62.6134, -44.2569.
\r\nIt was operated by the Intergalactic Naval Reserve Arm (INRA), a co-operative between the Federation and Empire that operated during the last war with the Thargoids. This facility appears to have had connections to the project that created the mycoid used against the Thargoids, and for a time hosted the researcher who originally stumbled across the mycoid.
\r\n
\r\nThe facility contains a number of agricultural tents, habitation modules, and a communications tower. A couple of small tanks marked with the symbol for the mycoid weapon are present, and the ground is stained in a manner consistent with leakage of the agent.
\r\n
\r\nThere are Engineer Materials scattered around the ground, and within the facility there are two Data Points which may be scanned for an Intel Package, and for Engineer Data; both need to be scanned within 20s.
\r\nAround the facility there are four Settlement Comms Log Uplink points, scanning them gives some of the history of the INRA:
\r\n\r\nWHISTLEBLOWER 1/4
\r\n\u2026Bypassing security protocols\u2026
\r\n\u2026Secure connection established\u2026
\r\nI don\u2019t have long. Once they realise I\u2019m gone and the data has been copied, they\u2019ll send their attack dogs after me, I know they will. But someone needs to show the galaxy what the INRA really is \u2013 what it\u2019s doing, what it\u2019s hiding.
\r\n\r\nWHISTLEBLOWER 2/4
\r\n\u2026Bypassing security protocols\u2026
\r\n\u2026Secure connection established\u2026
\r\nI\u2019m sorry for the part I\u2019ve played in this. Truly.
\r\nI was a researcher at a facility in the Hermitage 4 A system exploring agricultural applications of Thargoid-derived technology. The lab was owned by the INRA.
\r\nPublicly, the INRA likes to emphasise the whole altruistic and cooperative thing, but in recent years it\u2019s become much more focused on weapons testing and manufacture. Believe me, it\u2019s a military contractor in all but name.
\r\n\r\nWHISTLEBLOWER 3/4
\r\n\u2026Bypassing security protocols\u2026
\r\n\u2026Secure connection established\u2026
\r\nThe nature of my field was the study of disease-resistant crops and mycoproteins, that sort of thing. I was getting good results, even if my superiors took no notice. Then everything changed.
\r\nI was running a bunch of control experiments, just trying a few things out really. It was an afterthought \u2013 it wasn\u2019t even related to the main body of my work.
\r\nThe results were interesting and I didn\u2019t think they were particularly significant, but something made me take it directly to one of the INRA guys. I didn\u2019t want to go to Dr Prince, she\u2019d always been pretty dismissive of my work.
\r\nI would give anything to be able to undo that decision. Anything.
\r\n\r\nWHISTLEBLOWER 4/4
\r\n\u2026Bypassing security protocols\u2026
\r\n\u2026Secure connection established\u2026
\r\nAll my equipment and samples were whisked off to some remote facility. Later, I found out they\u2019d been taken to a weapons-testing site in the Alnath A 2 A A system.
\r\nMy research was used as the basis of a new super weapon designed to destroy the Thargoids. I heard they experimented on live captives. I doubt any of it was strictly legal. It certainly wasn\u2019t ethical.
\r\nTo the public, the INRA is a symbol of all that is possible when superpowers set aside their differences and work together. Well, it might have started off like that, but it\u2019s something very different now. Progress at any cost, might makes right \u2013 all our worst impulses channelled into an unaccountable organisation focused solely on making bigger and more powerful weapons. Bigger and more powerful weapons. God.
\r\nIf you find this\u2026 if someone finds this, make sure it gets out. Please. It\u2019s time people knew the truth.
\r\nThe logs contain references to the Hollis Gateway agricultural laboratory on Hermitage 4 a, and the Klatt Enterprises weapons-testing site on Alnath A 2 a a.
\r\n
"
+ },
+ {
+ "category": "Guardian",
+ "entry_name": "Soontill",
+ "system": "Soontill",
+ "x": 249.65625,
+ "y": -45.34375,
+ "z": 120,
+ "img": "img/soontill.png",
+ "html": "Soontill is an uninhabited system in the Core Systems within the Inner Orion Spur. It is allegedly home to a planet of the same name that was discovered by The Dark Wheel and is said to be replete with countless Thargoid treasures. The brothers Neptune and Oberon Ryder, sons of The Dark Wheel's leader, Alex Ryder, were originally divided over whether to pursue Raxxla or Soontill and eventually discovered the latter. Despite the location of the system Soontill being known and unrestricted, the planet's whereabouts remain a mystery to all but The Dark Wheel.
Objects taken from Soontill include slabs of unidentified material covered in pictographs of unknown origin. They are exclusively sold at Cheranovsky City in the Ngurii system as the Rare Commodity Soontill Relics. These alien artefacts were confirmed by Chief Xeno-Chemist Lyran Betar to not have been made by human hands and date back tens of thousands of years.
Tourist Spot 0508 describes a base in the Soontill system where a secret Human-Thargoid project occurred.
This is rumoured to be the site of the CIEP base. Some stories claim a combined Human-Thargoid project took place here.
The acronym CIEP could mean Circle of Independent Elite Pilots, an offshoot of The Dark Wheel.They used it as a secret military base before being run off by pirates with fancy military technology. The location of Soontill was found by the pirates because it was close to Grandmort and the origin of the Circle attack force which traveled (out of their way) through Brohman and Lanaest to reach Delta Phoenicis.
The location of Soontill given by Universal Cartographics may not be the actual place, because neither it nor Ngurii are close to the Grandmort system.
"
+ },
+ {
+ "category": "INRA",
+ "entry_name": "Carmichael Point",
+ "system": "HIP 16824",
+ "x": "-49.21875",
+ "y": "-89.37500",
+ "z": "-187.18750",
+ "img": "https://cdn-5f3c0a05c1ac180394b62f3c.closte.com/wp-content/uploads/2017/12/Screenshot_0073.png",
+ "html": "Carmichael Point is an abandoned settlement on HIP 16824 A 2 f at 73.8762, 61.8761.
\r\nThe location of this facility was given by an Unregistered Comms Beacon at HR 1188 A 2. The comms beacon transmits a message in phonetic alphabet on the hour.
\r\nSecure 9 14 18 1 Bait Deployed Contact Confirmed HIP 16824 A 2 F Secure
\r\n9 14 18 1 are the letter offsets for INRA
\r\nCarmichael Point was operated by the Intergalactic Naval Reserve Arm (INRA), a co-operative between the Federation and Empire that operated during the last war with the Thargoids. This facility appears to have had connections to the project that created the mycoid used against the Thargoids, it was used to lure a Thargoid ship in order to attack it. The attack failed, so happened presumably before the attack made by Commander Jameson.
\r\nThe facility contains a number of habitation modules, hangar or barracks buildings, and has two large mycoid storage tanks. There are collectable materials scattered around the site.
\r\nWithin the facility there is a Data Point which may be scanned for an Intel Package, and for Engineer Data. The Data Point also will give you the waypoint for the site in the Navigation Panel.
\r\nAround the facility there are four Settlement Comms Log Uplink points, scanning them gives some of the history of the INRA experiments:
"
+ }
+]
\ No newline at end of file
diff --git a/data/csvCache/route_UIA_Hyperdictions.csv b/data/csvCache/route_UIA_Hyperdictions.csv
new file mode 100644
index 0000000..051dd2e
--- /dev/null
+++ b/data/csvCache/route_UIA_Hyperdictions.csv
@@ -0,0 +1,2925 @@
+Timestamp,Commander,System,Sx,Sy,Sz,Destination,Dx,Dy,Dz,Jump Distance,Hostile
+2022-08-30 18:38:57,Mass1nhibited,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-08-30 18:57:13,FULGEN,Wolf 1279,"-51,46875","-117,46875","-250,78125",HIP 17481,"-58,84375","-150,25","-305,53125","64,2",
+2022-08-30 19:19:42,EmulatedPenguin,Pleiades Sector CH-L b8-2,"-102,78125","-138,75",-261,Pleiades Sector WP-O b6-2,"-103,0625",-159,"-295,3125","39,8",
+2022-08-30 19:22:09,EmulatedPenguin,Pleiades Sector CH-L b8-2,"-102,78125","-138,75",-261,Pleiades Sector WP-O b6-2,"-103,0625",-159,"-295,3125","39,8",
+2022-08-30 19:23:55,EmulatedPenguin,Pleiades Sector WP-O b6-2,"-103,0625",-159,"-295,3125",Pleiades Sector OD-S b4-2,"-90,28125","-154,3125","-332,03125","39,2",
+2022-08-30 19:25:39,EmulatedPenguin,Pleiades Sector WP-O b6-2,"-103,0625",-159,"-295,3125",Pleiades Sector OD-S b4-2,"-90,28125","-154,3125","-332,03125","39,2",
+2022-08-30 19:27:37,EmulatedPenguin,Pleiades Sector OD-S b4-2,"-90,28125","-154,3125","-332,03125",Pleiades Sector IM-V b2-3,"-88,90625","-168,25","-367,8125","38,4",
+2022-08-30 19:29:07,EmulatedPenguin,Pleiades Sector OD-S b4-2,"-90,28125","-154,3125","-332,03125",Pleiades Sector IM-V b2-3,"-88,90625","-168,25","-367,8125","38,4",
+2022-08-30 19:37:29,Node_,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-08-30 19:39:09,LilacLight,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-08-30 19:42:51,LilacLight,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-08-30 19:45:12,LilacLight,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-08-30 19:55:08,Galactik,HIP 22410,"-49,46875","-55,25","-360,59375",HIP 22460,"-41,3125","-58,96875","-354,78125","10,7",
+2022-08-30 20:03:22,Xarionn,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-08-30 20:05:01,Wotherspoon,Col 285 Sector DP-Y b1-4,"-30,46875","-58,625","-305,90625",Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875","57,6",
+2022-08-30 20:09:08,Xarionn,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-08-30 20:09:56,CodexNecro81,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-08-30 20:18:26,Mallchad,Pleiades Sector KC-U b3-1,"-62,40625","-133,0625","-360,875",HIP 19363,"-46,75","-117,75","-317,1875","48,9",
+2022-08-30 20:26:23,LilacLight,Oochorrs SU-K c10-0,"662,0625",-404,"-1865,5625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,5",
+2022-08-30 20:29:05,LilacLight,Oochorrs SU-K c10-0,"662,0625",-404,"-1865,5625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,5",
+2022-08-30 20:32:30,DaftMav,Sterope II,"-81,65625","-147,28125","-340,84375",Pleiades Sector HR-W d1-42,"-70,1875","-150,21875","-343,84375","12,2",
+2022-08-30 20:42:45,LilacLight,Oochorrs XA-J c11-0,"720,71875","-398,875","-1848,75",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","46,3",
+2022-08-30 20:43:27,LilacLight,Oochorrs XA-J c11-0,"720,71875","-398,875","-1848,75",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","46,3",
+2022-08-30 20:44:59,LilacLight,Oochorrs XA-J c11-0,"720,71875","-398,875","-1848,75",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","46,3",
+2022-08-30 20:45:34,DaftMav,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Pleiades Sector DL-Y d65,"-83,28125","-151,40625","-347,1875",14,
+2022-08-30 20:53:06,DaftMav,Pleiades Sector IH-V c2-7,"-78,46875","-144,9375","-324,9375",Pleiades Sector UO-Q b5-3,"-20,78125","-144,125","-323,59375","57,7",
+2022-08-30 21:43:10,APOC_V,Hyades Sector LN-Z a1-3,"-52,96875","-44,40625","-259,75",Col 285 Sector CP-Y b1-3,"-45,96875","-55,75","-324,625","66,2",
+2022-08-30 21:49:59,badloop,Oochorrs PO-M c9-0,"697,25","-420,0625","-1942,34375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","120,2",
+2022-08-30 23:03:20,Denis Page,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-08-30 23:04:49,Denis Page,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-08-30 23:04:58,Denis Page,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-08-30 23:05:18,Denis Page,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-08-30 23:08:10,h4cky,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-08-30 23:09:54,Radiumio,Oochorrs SU-K c10-0,"662,0625",-404,"-1865,5625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,5",
+2022-08-30 23:28:30,Daest,Oochorrs QZ-K c10-0,"659,6875","-384,78125","-1900,09375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","73,7",
+2022-08-30 23:28:56,Sebastian11220,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-08-30 23:34:35,Epaphus,Oochorrs AX-F c13-0,"689,1875","-344,9375","-1773,71875",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",65,
+2022-08-30 23:59:31,Sebastian11220,Oochorrs AH-H c12-0,"655,3125","-397,5625",-1802,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","49,8",
+2022-08-31 00:30:45,Molan Ryke,Oochorrs DS-F c13-0,"698,75","-361,34375","-1782,59375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","52,6",
+2022-08-31 01:14:12,Firehawk894,Oochorrs AH-H c12-0,"655,3125","-397,5625",-1802,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","49,8",
+2022-08-31 01:29:37,T'Verez,Oochorrs PT-P d6-0,667,"-418,15625","-1772,5",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","77,5",
+2022-08-31 01:57:49,h4cky,Oochorrs XA-J c11-0,"720,71875","-398,875","-1848,75",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","46,3",
+2022-08-31 02:00:54,h4cky,Oochorrs XA-J c11-0,"720,71875","-398,875","-1848,75",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","46,3",
+2022-08-31 02:56:48,JaCoVa,HIP 22410,"-49,46875","-55,25","-360,59375",Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875","5,6",
+2022-08-31 03:06:35,JaCoVa,Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875",HIP 22410,"-49,46875","-55,25","-360,59375","5,6",
+2022-08-31 04:03:19,Buur,Atlas,"-76,71875","-147,34375","-344,4375",Merope,"-78,59375","-149,625","-340,53125","4,9",
+2022-08-31 04:49:35,Kezika,Oochorrs AH-H c12-0,"655,3125","-397,5625",-1802,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","49,8",
+2022-08-31 05:44:22,bagofholding,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-08-31 07:14:45,T'Verez,Oochorrs HH-T d4-5,"723,40625","-404,625","-1880,25",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","68,5",
+2022-08-31 07:16:25,T'Verez,Oochorrs HH-T d4-5,"723,40625","-404,625","-1880,25",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","68,5",
+2022-08-31 07:21:34,bagofholding,Oochorrs AM-H c12-0,"735,8125","-383,21875","-1821,9375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,8",
+2022-08-31 07:35:35,Ex Bendible,Witch Head Sector GR-W d1-9,"331,625","-348,65625","-653,4375",Witch Head Sector KC-V c2-1,"342,59375","-393,5625","-695,9375","62,8",
+2022-08-31 07:39:38,GCSpitfire,Oochorrs DS-F c13-0,"698,75","-361,34375","-1782,59375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","52,6",
+2022-08-31 09:24:01,Molan Ryke,Oochorrs SU-K c10-0,"662,0625",-404,"-1865,5625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,5",
+2022-08-31 09:25:54,Molan Ryke,Oochorrs SU-K c10-0,"662,0625",-404,"-1865,5625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,5",
+2022-08-31 09:39:10,Dantechnik,Pleiades Sector CG-X c1-12,"-65,0625","-101,03125","-348,53125",Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875","46,7",
+2022-08-31 09:54:31,APOC_V,Oochorrs DS-F c13-0,"698,75","-361,34375","-1782,59375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","52,6",
+2022-08-31 09:55:43,APOC_V,Oochorrs DS-F c13-0,"698,75","-361,34375","-1782,59375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","52,6",
+2022-08-31 10:07:19,Charlene,Pleiades Sector HR-W d1-79,"-80,625","-146,65625","-343,25",Asterope,"-80,15625","-144,09375","-333,375","10,2",
+2022-08-31 10:21:14,Swoop Dogg,Hyades Sector ZU-Y c21,"-65,34375","-69,65625","-241,8125",Pleiades Sector KS-T c3-15,"-72,96875",-101,"-289,46875","57,5",
+2022-08-31 10:22:33,Hisaki51,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-08-31 10:27:16,Swoop Dogg,Pleiades Sector IH-V c2-7,"-78,46875","-144,9375","-324,9375",Asterope,"-80,15625","-144,09375","-333,375","8,6",
+2022-08-31 10:28:49,CP_Luiz,HIP 22711,"-35,75","-60,84375","-366,90625",Pleiades Sector DG-X c1-11,"-49,25","-70,4375","-360,8125","17,6",
+2022-08-31 10:39:51,LilacLight,Oochorrs AH-H c12-0,"655,3125","-397,5625",-1802,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","49,8",
+2022-08-31 10:42:03,LilacLight,Oochorrs AH-H c12-0,"655,3125","-397,5625",-1802,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","49,8",
+2022-08-31 10:58:58,LilacLight,Oochorrs BH-H c12-0,"698,9375","-422,21875","-1824,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,6",
+2022-08-31 11:00:55,LilacLight,Oochorrs BH-H c12-0,"698,9375","-422,21875","-1824,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,6",
+2022-08-31 11:01:25,LilacLight,Oochorrs BH-H c12-0,"698,9375","-422,21875","-1824,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,6",
+2022-08-31 11:06:54,Dantechnik,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-08-31 11:28:00,APOC_V,Oochorrs SU-K c10-0,"662,0625",-404,"-1865,5625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,5",
+2022-08-31 11:50:30,SturmWaffel,Oochorrs AH-H c12-0,"655,3125","-397,5625",-1802,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","49,8",
+2022-08-31 11:56:49,LilacLight,Oochorrs CH-H c12-0,"738,8125","-415,28125","-1821,09375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","68,6",
+2022-08-31 11:58:12,LilacLight,Oochorrs CH-H c12-0,"738,8125","-415,28125","-1821,09375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","68,6",
+2022-08-31 12:15:39,SturmWaffel,Oochorrs HH-T d4-5,"723,40625","-404,625","-1880,25",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","68,5",
+2022-08-31 12:16:52,SturmWaffel,Oochorrs HH-T d4-5,"723,40625","-404,625","-1880,25",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","68,5",
+2022-08-31 14:19:01,Hisaki51,Oochorrs AM-H c12-0,"735,8125","-383,21875","-1821,9375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,8",
+2022-08-31 15:55:00,Nebulov,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-08-31 16:17:56,Racius,HIP 23018,"-22,34375","-60,8125","-353,75",Taurus Dark Region YF-N b7-4,"-29,4375","-61,96875","-367,9375","15,9",
+2022-08-31 16:29:12,Marxanthius,Oochorrs AM-H c12-0,"735,8125","-383,21875","-1821,9375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,8",
+2022-08-31 16:39:17,L4F0r9e,Hyades Sector TD-B b2,"-29,34375","-66,40625","-272,6875",HR 1554,"-32,375","-57,53125","-340,65625","68,6",
+2022-08-31 17:20:21,Pete Justice,Taurus Dark Region WU-O b6-1,"-40,28125","-65,75","-385,90625",Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875","26,2",
+2022-08-31 17:25:40,Epaphus,Oochorrs AX-F c13-0,"689,1875","-344,9375","-1773,71875",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",65,
+2022-08-31 17:27:46,Epaphus,Oochorrs AX-F c13-0,"689,1875","-344,9375","-1773,71875",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",65,
+2022-08-31 17:32:14,Pete Justice,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875","8,4",
+2022-08-31 17:50:58,TheProRockPL,Oochorrs SU-K c10-0,"662,0625",-404,"-1865,5625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,5",
+2022-08-31 17:53:08,TheProRockPL,Oochorrs SU-K c10-0,"662,0625",-404,"-1865,5625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,5",
+2022-08-31 19:39:57,lAMOTHE,Synuefe QD-Z a68-0,"-41,8125","-43,9375","-329,40625",Synuefe QA-C b33-0,"-48,6875","-49,03125","-348,0625","20,5",
+2022-08-31 19:42:00,Phill P,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-08-31 19:57:41,Seven,Witch Head Sector SO-Q b5-0,"382,03125","-368,5625","-686,4375",HIP 23759,"359,84375","-385,53125","-718,375","42,4",
+2022-08-31 20:16:19,Lex Rottie,Taurus Dark Region TO-Q b5-2,"-5,96875","-69,5625","-407,28125",Synuefe WG-A b34-1,"-20,375","-62,0625","-338,5625","70,6",
+2022-08-31 20:18:20,Lex Rottie,Taurus Dark Region TO-Q b5-2,"-5,96875","-69,5625","-407,28125",Synuefe WG-A b34-1,"-20,375","-62,0625","-338,5625","70,6",
+2022-08-31 20:19:15,Lex Rottie,Taurus Dark Region TO-Q b5-2,"-5,96875","-69,5625","-407,28125",Synuefe WG-A b34-1,"-20,375","-62,0625","-338,5625","70,6",
+2022-08-31 20:25:52,kotie,Witch Head Sector YU-X b1-0,351,"-348,5","-780,6875",Witch Head Sector NI-S b4-0,"355,5",-373,"-717,125","68,3",
+2022-08-31 20:47:56,Lydia of the Void,Pleiades Sector AL-W b2-1,"-51,78125","-75,3125","-372,375",Pleiades Sector CW-U b3-3,"-50,5625","-63,53125","-362,5","15,4",
+2022-08-31 21:30:13,Stefanos,Oochorrs NI-R d5-1,"690,90625","-426,78125","-1804,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","60,9",
+2022-08-31 21:33:29,Historia-g,Oochorrs CC-H c12-0,"670,65625","-435,9375","-1804,09375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","70,8",
+2022-08-31 22:01:37,Argonaut Proxi,California Sector CL-Y d11,"-414,46875","-221,90625","-947,96875",California Sector EB-X c1-8,"-364,875","-224,03125","-911,75","61,4",
+2022-08-31 22:15:58,Suremaker,Oochorrs XA-J c11-0,"720,71875","-398,875","-1848,75",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","46,3",
+2022-08-31 22:42:17,EmulatedPenguin,Witch Head Sector TK-N b7-0,"316,125","-342,6875","-662,90625",Siniang,"354,40625","-386,625","-709,21875","74,4",
+2022-08-31 22:45:48,Nathan Kerbonaut,Oochorrs SU-K c10-0,"662,0625",-404,"-1865,5625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,5",
+2022-08-31 22:55:05,WiMpY Nux,HIP 23018,"-22,34375","-60,8125","-353,75",HIP 22460,"-41,3125","-58,96875","-354,78125","19,1",
+2022-08-31 23:07:41,VEGAMAX,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-08-31 23:11:29,M. V. Coehoorn,Oochorrs XA-J c11-0,"720,71875","-398,875","-1848,75",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","46,3",
+2022-08-31 23:28:39,CodexNecro81,Oochorrs YV-I c11-0,"684,84375","-428,40625","-1856,1875",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","60,4",
+2022-09-01 00:41:17,Marxanthius,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-09-01 02:17:40,FetalDave,Oochorrs DS-F c13-0,"698,75","-361,34375","-1782,59375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","52,6",
+2022-09-01 02:25:31,OverloadRJ45,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-09-01 03:15:58,Sayaka,Swoilz OO-X d2-10,"467,96875","-39,4375","468,28125",Musca Dark Region ZB-I a11-0,"425,90625","-3,75","267,5","208,2",
+2022-09-01 03:34:54,Helios Maximus,Oochorrs SU-K c10-0,"662,0625",-404,"-1865,5625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,5",
+2022-09-01 04:03:30,Jack Wilmslow,Maia,"-81,78125","-149,4375","-343,375",Atlas,"-76,71875","-147,34375","-344,4375","5,6",
+2022-09-01 04:04:44,Jack Wilmslow,Maia,"-81,78125","-149,4375","-343,375",Atlas,"-76,71875","-147,34375","-344,4375","5,6",
+2022-09-01 05:54:25,Verbotron,Synuefe CH-T d4-13,"301,65625","-364,3125","-618,875",Witch Head Sector UU-O b6-0,"336,4375","-382,40625","-679,625","72,3",
+2022-09-01 05:57:13,Verbotron,Synuefe CH-T d4-13,"301,65625","-364,3125","-618,875",Witch Head Sector UU-O b6-0,"336,4375","-382,40625","-679,625","72,3",
+2022-09-01 07:59:08,Lou Snockers,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-09-01 08:02:34,Acred,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-09-01 08:26:25,SharkBiscuit,HR 1185,"-64,65625","-148,9375","-330,40625",Delphi,"-63,59375","-147,40625","-319,09375","11,5",
+2022-09-01 08:29:21,SharkBiscuit,HR 1185,"-64,65625","-148,9375","-330,40625",Delphi,"-63,59375","-147,40625","-319,09375","11,5",
+2022-09-01 08:47:03,Namix,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-09-01 09:15:23,Grover Kiwi,Oochorrs AM-H c12-0,"735,8125","-383,21875","-1821,9375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,8",
+2022-09-01 09:53:42,winston george,Oochorrs PT-P d6-4,"696,625","-382,1875","-1758,03125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","75,7",
+2022-09-01 10:02:16,Lou Snockers,Oochorrs XA-J c11-0,"720,71875","-398,875","-1848,75",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","46,3",
+2022-09-01 11:57:42,LilacLight,Oochorrs WA-J c11-0,"658,09375","-423,84375","-1859,34375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","64,1",
+2022-09-01 12:16:27,CodexNecro81,Oochorrs YV-I c11-0,"684,84375","-428,40625","-1856,1875",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","60,4",
+2022-09-01 12:52:54,TheProRockPL,Oochorrs XA-J c11-0,"720,71875","-398,875","-1848,75",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","46,3",
+2022-09-01 12:53:49,psykit,Col 285 Sector CV-Y d42,"-21,21875","-51,53125","-310,53125",HR 1554,"-32,375","-57,53125","-340,65625","32,7",
+2022-09-01 12:58:51,psykit,HR 1554,"-32,375","-57,53125","-340,65625",HIP 22460,"-41,3125","-58,96875","-354,78125","16,8",
+2022-09-01 12:59:06,Grimscrub,Oochorrs XA-J c11-0,"720,71875","-398,875","-1848,75",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","46,3",
+2022-09-01 13:19:27,APOC_V,Oochorrs XA-J c11-0,"720,71875","-398,875","-1848,75",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","46,3",
+2022-09-01 13:20:52,psykit,HR 1554,"-32,375","-57,53125","-340,65625",HIP 22460,"-41,3125","-58,96875","-354,78125","16,8",
+2022-09-01 13:34:14,psykit,HR 1554,"-32,375","-57,53125","-340,65625",Pleiades Sector KS-S b4-2,"-50,84375","-86,40625","-326,4375","37,1",
+2022-09-01 13:48:10,Shadowspawn2,Witch Head Sector SZ-O b6-0,"341,4375","-354,0625","-679,96875",Shenve,"351,96875","-373,46875","-711,09375","38,2",
+2022-09-01 13:58:52,psykit,Maia,"-81,78125","-149,4375","-343,375",Celaeno,"-81,09375","-148,3125","-337,09375","6,4",
+2022-09-01 14:35:23,psykit,Col 285 Sector HV-W b2-2,"-31,59375","-45,375","-297,65625",Synuefe KD-A c17-10,"-32,375","-58,1875","-332,6875","37,3",
+2022-09-01 14:38:16,psykit,Synuefe KD-A c17-10,"-32,375","-58,1875","-332,6875",HR 1554,"-32,375","-57,53125","-340,65625",8,
+2022-09-01 14:38:21,diocletian valerius,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-09-01 14:40:52,diocletian valerius,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-09-01 14:56:36,diocletian valerius,Oochorrs SU-K c10-0,"662,0625",-404,"-1865,5625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,5",
+2022-09-01 15:08:43,Helmut Grokenberger,Oochorrs DS-F c13-0,"698,75","-361,34375","-1782,59375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","52,6",
+2022-09-01 17:38:37,Tytonid,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","66,6",
+2022-09-01 17:40:01,Tytonid,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","66,6",
+2022-09-01 17:48:46,DarknessCs101,Oochorrs SU-K c10-0,"662,0625",-404,"-1865,5625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,5",
+2022-09-01 18:01:00,csn,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-01 18:21:41,Moscito,HIP 23759,"359,84375","-385,53125","-718,375",Shenve,"351,96875","-373,46875","-711,09375","16,1",
+2022-09-01 18:22:40,AXELANDRE,Oochorrs SU-K c10-0,"662,0625",-404,"-1865,5625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,5",
+2022-09-01 18:24:24,AXELANDRE,Oochorrs SU-K c10-0,"662,0625",-404,"-1865,5625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,5",
+2022-09-01 18:24:50,CodexNecro81,Oochorrs YV-I c11-0,"684,84375","-428,40625","-1856,1875",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","60,4",
+2022-09-01 18:25:05,Mallchad,Oochorrs XA-J c11-0,"720,71875","-398,875","-1848,75",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","46,3",
+2022-09-01 18:25:50,Mallchad,Oochorrs XA-J c11-0,"720,71875","-398,875","-1848,75",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","46,3",
+2022-09-01 18:26:10,CodexNecro81,Oochorrs YV-I c11-0,"684,84375","-428,40625","-1856,1875",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","60,4",
+2022-09-01 18:26:43,Mallchad,Oochorrs XA-J c11-0,"720,71875","-398,875","-1848,75",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","46,3",
+2022-09-01 18:27:03,Mallchad,Oochorrs XA-J c11-0,"720,71875","-398,875","-1848,75",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","46,3",
+2022-09-01 18:37:52,Zer0Axis,Oochorrs DS-F c13-0,"698,75","-361,34375","-1782,59375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","52,6",
+2022-09-01 18:43:53,LilacLight,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-01 18:46:03,LilacLight,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-01 19:01:22,Mallchad,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-01 19:07:09,Kell Adair,Atlas,"-76,71875","-147,34375","-344,4375",Asterope,"-80,15625","-144,09375","-333,375",12,
+2022-09-01 19:10:53,Mallchad,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-01 19:14:45,JaCoVa,Asterope,"-80,15625","-144,09375","-333,375",Sterope II,"-81,65625","-147,28125","-340,84375","8,3",
+2022-09-01 20:19:07,Wallywest,Synuefe UG-A b34-1,"-54,3125","-51,375","-331,375",HIP 22460,"-41,3125","-58,96875","-354,78125","27,8",
+2022-09-01 20:25:07,LilacLight,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-01 20:26:05,LilacLight,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-01 20:54:09,pokegustavo,Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875",HIP 22460,"-41,3125","-58,96875","-354,78125","8,4",
+2022-09-01 20:59:19,Yularen Mapi's,Oochorrs AH-H c12-0,"655,3125","-397,5625",-1802,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","49,8",
+2022-09-01 21:00:17,Yularen Mapi's,Oochorrs AH-H c12-0,"655,3125","-397,5625",-1802,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","49,8",
+2022-09-01 21:23:33,PsydaCanns,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-09-01 21:38:12,Unknown Zombie,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-01 21:39:46,Unknown Zombie,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-01 21:40:12,Unknown Zombie,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-01 21:43:20,pokegustavo,Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875",HIP 22460,"-41,3125","-58,96875","-354,78125","8,4",
+2022-09-01 21:55:47,Argonaut Proxi,California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875",California Sector LX-T b3-0,"-337,90625","-211,9375","-927,03125","27,1",
+2022-09-01 22:19:18,pokegustavo,Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875",HIP 22460,"-41,3125","-58,96875","-354,78125","8,4",
+2022-09-01 22:44:40,pokegustavo,Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875",HIP 22460,"-41,3125","-58,96875","-354,78125","8,4",
+2022-09-01 23:13:41,EmulatedPenguin,Pleiades Sector OX-T b3-0,"-19,375","-150,15625","-354,1875",HIP 18502,"-55,1875","-147,71875","-341,09375","38,2",
+2022-09-01 23:15:34,EmulatedPenguin,Pleiades Sector OX-T b3-0,"-19,375","-150,15625","-354,1875",HIP 18502,"-55,1875","-147,71875","-341,09375","38,2",
+2022-09-01 23:29:28,Oldcarsmell,Pleiades Sector ER-U b3-2,"-52,875","-77,71875","-349,375",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","18,6",
+2022-09-01 23:36:26,pokegustavo,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875","8,4",
+2022-09-01 23:49:42,Finnanator252,Oochorrs TF-J c11-0,"617,65625","-368,5625","-1838,0625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","68,8",
+2022-09-02 01:30:39,JaCoVa,Sterope II,"-81,65625","-147,28125","-340,84375",Asterope,"-80,15625","-144,09375","-333,375","8,3",
+2022-09-02 01:31:44,JaCoVa,Sterope II,"-81,65625","-147,28125","-340,84375",Asterope,"-80,15625","-144,09375","-333,375","8,3",
+2022-09-02 01:39:10,EmulatedPenguin,Pleiades Sector MS-T b3-1,-104,-180,"-358,75",Pleiades Sector GM-V b2-0,"-135,125","-173,5","-379,96875","38,2",
+2022-09-02 01:45:12,EmulatedPenguin,Pleiades Sector MS-T b3-1,-104,-180,"-358,75",Pleiades Sector GM-V b2-0,"-135,125","-173,5","-379,96875","38,2",
+2022-09-02 01:59:19,Sayaka,Synuefe MX-A a68-3,"-36,71875","-41,71875","-335,75",HIP 22460,"-41,3125","-58,96875","-354,78125","26,1",
+2022-09-02 02:06:44,LilacLight,Oochorrs SU-K c10-0,"662,0625",-404,"-1865,5625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,5",
+2022-09-02 02:07:07,Sayaka,HIP 22460,"-41,3125","-58,96875","-354,78125",Synuefe GX-B c16-6,"-37,25","-55,125","-347,15625","9,5",
+2022-09-02 02:09:11,LilacLight,Oochorrs SU-K c10-0,"662,0625",-404,"-1865,5625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,5",
+2022-09-02 02:17:09,Sayaka,HIP 22460,"-41,3125","-58,96875","-354,78125",Synuefe IR-C a67-1,-42,"-44,28125","-354,3125","14,7",
+2022-09-02 02:25:27,CP_Luiz,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-02 02:26:58,CP_Luiz,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-02 02:38:59,CP_Luiz,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-02 03:53:34,CP_Luiz,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-02 04:06:32,Rogixd,Witch Head Sector EB-W b2-0,"375,15625","-359,3125","-759,21875",Shenve,"351,96875","-373,46875","-711,09375","55,3",
+2022-09-02 04:57:34,Marko S Ramius,Oochorrs DS-F c13-0,"698,75","-361,34375","-1782,59375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","52,6",
+2022-09-02 04:59:04,Marko S Ramius,Oochorrs DS-F c13-0,"698,75","-361,34375","-1782,59375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","52,6",
+2022-09-02 09:19:49,Marko S Ramius,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-02 11:43:26,Elrith,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22711,"-35,75","-60,84375","-366,90625","17,8",
+2022-09-02 14:55:53,L4F0r9e,Oochorrs XA-J c11-0,"720,71875","-398,875","-1848,75",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","46,3",
+2022-09-02 15:06:58,CJMVAUCLUSE,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-09-02 15:48:07,Supergurke,Oochorrs VQ-H c12-0,"617,25","-343,21875","-1819,59375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","76,1",
+2022-09-02 15:49:45,Supergurke,Oochorrs VQ-H c12-0,"617,25","-343,21875","-1819,59375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","76,1",
+2022-09-02 15:50:27,Supergurke,Oochorrs VQ-H c12-0,"617,25","-343,21875","-1819,59375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","76,1",
+2022-09-02 16:00:45,Supergurke,Oochorrs VQ-H c12-0,"617,25","-343,21875","-1819,59375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","76,1",
+2022-09-02 16:22:06,allan mandragoran,Witch Head Sector AF-Z b0,"381,59375","-401,375","-796,8125",Witch Head Sector GW-W c1-5,"348,34375","-420,0625",-722,84,
+2022-09-02 16:51:55,ODSKEE,Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875",Pleiades Sector ER-U b3-2,"-52,875","-77,71875","-349,375","22,1",
+2022-09-02 17:00:14,Toqueville,Pleiades Sector FR-V c2-3,"-58,1875",-65,"-336,9375",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","20,2",
+2022-09-02 17:00:15,Dave Weyland,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-09-02 17:09:51,Toqueville,Pleiades Sector FR-V c2-3,"-58,1875",-65,"-336,9375",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","20,2",
+2022-09-02 17:19:56,Icho Tolt,Musca Dark Region HM-V c2-21,"443,78125","-3,84375","294,0625",HIP 62359,"445,375","0,15625","286,3125","8,9",
+2022-09-02 17:26:24,Squeesquee,Struve's Lost Sector CB-N b7-2,"24,90625","-155,6875","-419,6875",HIP 19669,"-32,0625","-150,46875","-372,6875",74,
+2022-09-02 17:31:45,ODSKEE,Pleiades Sector NI-S b4-2,"-66,4375","-131,375","-336,21875",HR 1183,"-72,875","-145,09375","-336,96875","15,2",
+2022-09-02 17:52:07,Toqueville,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",
+2022-09-02 18:14:31,Bim,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-09-02 18:14:52,Bim,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-09-02 18:34:06,Soundo,HIP 21852,"-38,53125","-75,3125","-352,40625",HIP 22460,"-41,3125","-58,96875","-354,78125","16,7",
+2022-09-02 18:42:00,NSR2,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-09-02 19:04:40,Soundo,HIP 22460,"-41,3125","-58,96875","-354,78125",HIP 21852,"-38,53125","-75,3125","-352,40625","16,7",
+2022-09-02 19:34:43,Kira Nakatani,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","45,2",
+2022-09-02 19:41:38,Gaesatae,California Sector XO-A c2,"-342,40625","-212,34375","-990,53125",California Sector CV-Y c4,"-304,78125","-215,03125","-947,34375","57,3",
+2022-09-02 19:58:15,HunabKU,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-02 20:00:42,HunabKU,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-02 20:27:37,Eckee,Oochorrs TF-J c11-0,"617,65625","-368,5625","-1838,0625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","68,8",
+2022-09-02 20:45:40,Eckee,Oochorrs TF-J c11-0,"617,65625","-368,5625","-1838,0625",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","68,8",
+2022-09-02 20:46:35,PanPiper,Oochorrs AH-H c12-0,"655,3125","-397,5625",-1802,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","49,8",
+2022-09-02 20:50:22,Novaseta,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-02 21:14:17,PanPiper,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-02 21:23:24,nyzchf,HIP 17403,"-93,6875","-158,96875","-367,625",Pleiades Sector IH-V c2-5,"-78,71875","-138,03125","-321,78125","52,6",
+2022-09-02 21:28:14,Novaseta,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-02 21:31:18,Novaseta,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-02 21:36:57,Toqueville,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","5,2",
+2022-09-02 21:40:35,Stefanos,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22711,"-35,75","-60,84375","-366,90625","17,8",
+2022-09-02 21:52:30,Novaseta,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-02 21:55:18,Novaseta,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-02 22:35:03,Rogixd,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-02 22:41:40,Rogixd,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-02 22:51:32,Wotherspoon,Siniang,"354,40625","-386,625","-709,21875",Witch Head Sector GW-W c1-1,"346,15625","-411,21875","-710,6875",26,
+2022-09-02 22:59:48,Wotherspoon,Evangelis,"353,75","-419,03125",-710,Witch Head Sector KC-V c2-4,"360,84375","-396,96875","-704,6875","23,8",
+2022-09-02 23:30:23,Alfredo Cavatelli,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-02 23:30:53,SIX-SHOOTER,Oochorrs DS-F c13-0,"698,75","-361,34375","-1782,59375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","52,6",
+2022-09-03 00:15:20,pokegustavo,Synuefe VG-A b34-4,-39,"-49,9375","-335,9375",Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875","28,3",
+2022-09-03 00:20:41,pokegustavo,Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","9,9",
+2022-09-03 00:23:29,pokegustavo,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",
+2022-09-03 01:22:43,rotas miller,Synuefe VG-A b34-4,-39,"-49,9375","-335,9375",HIP 22460,"-41,3125","-58,96875","-354,78125",21,
+2022-09-03 01:43:01,pokegustavo,Hyades Sector RI-B b1,"-37,4375","-55,1875","-269,53125",Pleiades Sector GW-W d1-33,"-50,25","-62,8125","-326,8125","59,2",
+2022-09-03 01:55:40,pokegustavo,Pleiades Sector GW-W d1-33,"-50,25","-62,8125","-326,8125",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","25,4",
+2022-09-03 02:05:35,Djbeef,Pleiades Sector RO-Q b5-3,"-70,46875","-131,8125","-306,78125",Delphi,"-63,59375","-147,40625","-319,09375",21,
+2022-09-03 04:44:52,ShoggothPete,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-03 05:24:28,BL1P,Oochorrs XA-J c11-0,"720,71875","-398,875","-1848,75",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","46,3",
+2022-09-03 05:35:21,BL1P,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-03 06:36:48,Ex Bendible,Oochorrs AM-H c12-0,"735,8125","-383,21875","-1821,9375",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","51,8",
+2022-09-03 09:50:23,Mass1nhibited,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector KH-V c2-1,"-296,78125","-211,78125","-886,5","35,8",
+2022-09-03 09:57:36,Poperrap,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-03 12:07:29,GCSpitfire,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375","57,2",
+2022-09-03 12:23:12,Squeesquee,Taurus Dark Region NA-B a15-2,"-72,28125","-28,6875","-380,375",Taurus Dark Region GW-W d1-74,"-58,8125","-44,15625","-363,78125","26,4",
+2022-09-03 12:25:28,Squeesquee,Taurus Dark Region GW-W d1-74,"-58,8125","-44,15625","-363,78125",HIP 22460,"-41,3125","-58,96875","-354,78125","24,6",
+2022-09-03 12:41:11,Grimscrub,Sterope II,"-81,65625","-147,28125","-340,84375",Pleiades Sector EB-X c1-3,"-65,125","-109,875","-358,46875","44,5",
+2022-09-03 12:44:00,Grimscrub,Pleiades Sector EB-X c1-3,"-65,125","-109,875","-358,46875",Pleiades Sector DG-X c1-10,"-53,34375","-66,8125","-357,28125","44,7",
+2022-09-03 12:55:32,Eckee,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-03 12:57:50,ProxyAlpha,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625","10,5",
+2022-09-03 13:03:39,Mass1nhibited,California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","24,3",
+2022-09-03 13:10:57,ProxyAlpha,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-03 13:11:15,ProxyAlpha,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-03 13:13:16,Mallchad,Oochorrs IT-D c14-0,"657,40625","-423,34375","-1739,875",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","58,6",
+2022-09-03 13:29:38,Carsie,Pleiades Sector HR-W d1-74,"-87,40625","-150,90625","-344,96875",Pleiades Sector KC-V c2-10,"-87,3125","-149,90625","-343,46875","1,8",
+2022-09-03 13:31:26,Carsie,Pleiades Sector HR-W d1-74,"-87,40625","-150,90625","-344,96875",Pleiades Sector KC-V c2-10,"-87,3125","-149,90625","-343,46875","1,8",
+2022-09-03 13:33:55,Mass1nhibited,California Sector LC-V c2-4,"-318,34375","-230,75","-888,21875",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375","10,1",
+2022-09-03 13:34:50,Carsie,Pleiades Sector KC-V c2-10,"-87,3125","-149,90625","-343,46875",Pleiades Sector HR-W d1-74,"-87,40625","-150,90625","-344,96875","1,8",
+2022-09-03 13:44:57,Carsie,HR 1183,"-72,875","-145,09375","-336,96875",HIP 17692,"-74,125","-143,96875","-328,78125","8,4",
+2022-09-03 13:59:05,Mallchad,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-03 14:22:17,LilacLight,Oochorrs ED-E c14-0,"658,1875","-343,28125","-1739,25",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","60,3",
+2022-09-03 14:40:27,LilacLight,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-03 14:57:18,LilacLight,Oochorrs AH-H c12-0,"655,3125","-397,5625",-1802,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",23,
+2022-09-03 14:58:57,LilacLight,Oochorrs AH-H c12-0,"655,3125","-397,5625",-1802,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",23,
+2022-09-03 15:27:01,LilacLight,Oochorrs DN-F c13-0,"647,25","-407,53125","-1761,34375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","34,1",
+2022-09-03 15:29:03,LilacLight,Oochorrs DN-F c13-0,"647,25","-407,53125","-1761,34375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","34,1",
+2022-09-03 15:32:41,Seph Iridan,Oochorrs AH-H c12-0,"655,3125","-397,5625",-1802,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",23,
+2022-09-03 15:38:27,Requese,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-03 15:48:10,BlakeA,Pleione,-77,"-146,78125","-344,125",Pleiades Sector HR-W d1-74,"-87,40625","-150,90625","-344,96875","11,2",
+2022-09-03 16:02:32,LilacLight,Oochorrs GY-D c14-0,"672,6875","-346,84375","-1726,875",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","69,3",
+2022-09-03 16:29:54,Requese,Oochorrs GY-D c14-0,"672,6875","-346,84375","-1726,875",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","69,3",
+2022-09-03 16:33:41,SQUIDGOD,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-03 16:35:05,SQUIDGOD,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-03 16:46:43,Chundaman,Synuefe GX-B c16-5,"-32,9375","-57,5625","-348,15625",HIP 21852,"-38,53125","-75,3125","-352,40625","19,1",
+2022-09-03 16:49:29,Chundaman,HIP 21852,"-38,53125","-75,3125","-352,40625",Pleiades Sector GM-U b3-0,"-48,375","-97,09375","-351,28125","23,9",
+2022-09-03 16:59:09,REBECA ASIMOV,Witch Head Sector OD-S b4-0,"340,84375","-400,28125","-706,84375",Ronemar,"355,75","-400,5","-707,21875","14,9",
+2022-09-03 17:26:37,Seph Iridan,Oochorrs MY-P d6-6,"629,53125","-340,5","-1776,125",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",53,
+2022-09-03 17:37:51,InvestigateXM,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-03 17:51:14,IvIePhisto,Pleiades Sector IX-S b4-0,"-61,25","-84,25","-341,875",Pleiades Sector CQ-Y d58,"-53,5625","-60,9375","-353,03125",27,
+2022-09-03 18:10:03,caRGOrunner1969,Pleiades Sector IH-V c2-6,"-77,625","-142,21875","-325,25",Asterope,"-80,15625","-144,09375","-333,375","8,7",
+2022-09-03 18:11:27,caRGOrunner1969,Pleiades Sector IH-V c2-6,"-77,625","-142,21875","-325,25",Asterope,"-80,15625","-144,09375","-333,375","8,7",
+2022-09-03 19:17:25,Emovolcrom,Pleiades Sector CG-X c1-12,"-65,0625","-101,03125","-348,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","48,7",
+2022-09-03 19:20:02,Emovolcrom,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","5,2",
+2022-09-03 20:07:53,Gaesatae,Coalsack Sector UF-N b7-9,"374,125","40,625","320,75",Musca Dark Region OJ-P b6-12,"408,84375","4,5625","276,59375","66,8",
+2022-09-03 20:23:56,OverloadRJ45,Witch Head Sector AK-Z b0,"417,875","-382,21875","-800,625",Witch Head Sector GW-V b2-0,"376,3125","-384,1875","-761,1875","57,3",
+2022-09-03 20:41:01,Grokwix,Oochorrs AH-H c12-0,"655,3125","-397,5625",-1802,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",23,
+2022-09-03 21:19:21,Rogixd,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-03 21:59:15,Rogixd,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-03 22:08:10,Rogixd,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-03 22:55:09,Transom,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-03 22:55:16,Transom,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-03 23:04:17,Eckee,Oochorrs DN-F c13-0,"647,25","-407,53125","-1761,34375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","34,1",
+2022-09-03 23:07:40,Transom,Oochorrs AH-H c12-0,"655,3125","-397,5625",-1802,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",23,
+2022-09-03 23:07:46,Transom,Oochorrs AH-H c12-0,"655,3125","-397,5625",-1802,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",23,
+2022-09-03 23:54:19,Rogixd,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-04 01:46:40,Emovolcrom,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","42,7",
+2022-09-04 04:50:26,PanPiper,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-04 04:50:39,PanPiper,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-04 07:00:51,67MistakeNot,Oochorrs AH-H c12-0,"655,3125","-397,5625",-1802,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",23,
+2022-09-04 07:41:32,BL1P,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-04 08:15:36,caRGOrunner1969,Asterope,"-80,15625","-144,09375","-333,375",Celaeno,"-81,09375","-148,3125","-337,09375","5,7",
+2022-09-04 09:01:05,caRGOrunner1969,Celaeno,"-81,09375","-148,3125","-337,09375",HR 1183,"-72,875","-145,09375","-336,96875","8,8",
+2022-09-04 09:02:14,caRGOrunner1969,Celaeno,"-81,09375","-148,3125","-337,09375",HR 1183,"-72,875","-145,09375","-336,96875","8,8",
+2022-09-04 09:10:59,pm3,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-04 09:12:02,pm3,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-04 09:12:13,pm3,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-04 09:12:46,pm3,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-04 09:14:00,pm3,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-04 09:18:28,pm3,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-04 09:34:30,HunabKU,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-04 09:47:22,HunabKU,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-04 10:24:12,kjnpbr,Pleiades Sector TZ-O b6-0,"-80,40625","-124,125","-304,125",Pleiades Sector HR-W d1-57,"-86,40625","-125,15625","-301,84375","6,5",
+2022-09-04 10:25:30,kjnpbr,Pleiades Sector TZ-O b6-0,"-80,40625","-124,125","-304,125",Pleiades Sector HR-W d1-57,"-86,40625","-125,15625","-301,84375","6,5",
+2022-09-04 10:28:29,TomCatT,Pleiades Sector WU-O b6-0,"-53,875","-128,15625","-297,71875",Delphi,"-63,59375","-147,40625","-319,09375","30,4",
+2022-09-04 10:30:31,Mass1nhibited,California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","24,3",
+2022-09-04 10:48:20,Mass1nhibited,California Sector LC-V c2-2,"-312,59375","-226,28125","-878,5",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375","12,8",
+2022-09-04 11:03:05,Seph Iridan,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-04 11:23:51,Rogixd,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-04 11:39:52,Rogixd,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-04 11:44:20,TomCatT,Delphi,"-63,59375","-147,40625","-319,09375",Pleiades Sector GW-W c1-15,"-76,9375","-153,71875","-346,625","31,2",
+2022-09-04 12:06:35,Hanna Hunter,California Sector DL-Y d2,"-320,46875","-204,1875","-925,53125",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","17,4",
+2022-09-04 12:11:54,Gargantuan Jockey,Oochorrs AH-H c12-0,"655,3125","-397,5625",-1802,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",23,
+2022-09-04 12:19:58,Rogixd,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-04 12:48:26,Rogixd,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-04 13:21:19,Powell Oblivion,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-04 13:25:50,Powell Oblivion,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-04 13:34:01,Hudiny,Col 285 Sector OC-T b4-5,"-104,84375","-83,75","-264,90625",Pleiades Sector XP-O b6-2,"-81,53125","-154,96875","-302,25","83,7",
+2022-09-04 13:35:30,Powell Oblivion,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-04 13:36:50,Powell Oblivion,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-04 13:38:26,Powell Oblivion,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625","10,5",
+2022-09-04 13:39:55,Firedrax,HR 1183,"-72,875","-145,09375","-336,96875",Pleiades Sector IW-V b2-3,"-17,46875",-129,"-374,1875","68,7",
+2022-09-04 14:33:16,Dantechnik,Witch Head Sector BQ-X b1-0,"359,28125",-385,"-782,46875",Witch Head Sector EB-X c1-8,"367,375","-379,1875","-734,9375","48,6",
+2022-09-04 14:35:45,Molan Ryke,Pleiades Sector CW-U b3-4,"-54,5","-64,875","-359,9375",HIP 22460,"-41,3125","-58,96875","-354,78125","15,3",
+2022-09-04 14:44:03,Powell Oblivion,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625","10,5",
+2022-09-04 14:48:59,Gray Z7Z,Pleiades Sector PD-S b4-2,"-75,5625","-152,40625","-336,40625",Asterope,"-80,15625","-144,09375","-333,375",10,
+2022-09-04 14:52:17,Hurix,Oochorrs AH-H c12-0,"655,3125","-397,5625",-1802,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",23,
+2022-09-04 14:52:20,Powell Oblivion,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625","10,5",
+2022-09-04 14:55:54,Gray Z7Z,Pleiades Sector PD-S b4-2,"-75,5625","-152,40625","-336,40625",Asterope,"-80,15625","-144,09375","-333,375",10,
+2022-09-04 14:57:12,Powell Oblivion,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-04 15:53:49,Powell Oblivion,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",N
+2022-09-04 15:58:01,BIA RoS,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875","8,4",
+2022-09-04 15:59:29,Powell Oblivion,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",N
+2022-09-04 16:00:10,Kell Adair,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","42,7",
+2022-09-04 16:00:18,Volrathu,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-04 16:00:29,Helmut Grokenberger,Oochorrs MY-P d6-6,"629,53125","-340,5","-1776,125",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",53,
+2022-09-04 16:01:04,BIA RoS,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875","8,4",
+2022-09-04 16:02:00,Kell Adair,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","42,7",
+2022-09-04 16:02:20,Powell Oblivion,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625","10,5",Y
+2022-09-04 16:02:33,BIA RoS,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875","8,4",
+2022-09-04 16:20:47,Alcer,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-04 16:20:54,GCSpitfire,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625","10,5",
+2022-09-04 16:37:38,BIA RoS,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",Pleiades Sector CQ-Y d58,"-53,5625","-60,9375","-353,03125","8,9",
+2022-09-04 16:40:11,BIA RoS,Pleiades Sector CQ-Y d58,"-53,5625","-60,9375","-353,03125",Pleiades Sector DG-X c1-10,"-53,34375","-66,8125","-357,28125","7,3",
+2022-09-04 17:00:09,Vegassimus,Oochorrs DN-F c13-0,"647,25","-407,53125","-1761,34375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","34,1",
+2022-09-04 17:07:39,pokegustavo,Pleiades Sector HC-T b4-0,"-43,25","-60,03125","-343,03125",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","8,7",
+2022-09-04 17:13:04,pokegustavo,Pleiades Sector HC-T b4-0,"-43,25","-60,03125","-343,03125",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","8,7",
+2022-09-04 17:19:20,Theory Madness,Oochorrs CC-H c12-0,"670,65625","-435,9375","-1804,09375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","56,9",
+2022-09-04 17:21:09,Theory Madness,Oochorrs CC-H c12-0,"670,65625","-435,9375","-1804,09375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","56,9",
+2022-09-04 17:29:42,Eckee,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-04 18:26:41,Space_Trash,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-04 18:35:03,PreciselyTuned,Oochorrs AH-H c12-0,"655,3125","-397,5625",-1802,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625","29,3",N
+2022-09-04 18:35:56,SQUIDGOD,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-04 18:37:12,SQUIDGOD,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-04 18:37:31,SQUIDGOD,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-04 18:43:47,Gray Z7Z,Pleiades Sector PD-S b4-2,"-75,5625","-152,40625","-336,40625",HR 1172,"-75,1875","-152,40625","-346,59375","10,2",
+2022-09-04 19:04:48,Gray Z7Z,HR 1172,"-75,1875","-152,40625","-346,59375",Pleiades Sector PD-S b4-2,"-75,5625","-152,40625","-336,40625","10,2",
+2022-09-04 19:26:32,pokegustavo,Hyades Sector RI-B b1,"-37,4375","-55,1875","-269,53125",Pleiades Sector GW-W d1-33,"-50,25","-62,8125","-326,8125","59,2",
+2022-09-04 20:29:41,Stefanos,HIP 22711,"-35,75","-60,84375","-366,90625",HIP 22460,"-41,3125","-58,96875","-354,78125","13,5",N
+2022-09-04 20:37:45,Stefanos,HIP 22711,"-35,75","-60,84375","-366,90625",HIP 22460,"-41,3125","-58,96875","-354,78125","13,5",N
+2022-09-04 21:21:38,Hisaki51,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-04 21:23:36,Hisaki51,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-04 21:34:00,Hisaki51,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-04 22:22:34,GCSpitfire,Oochorrs DN-F c13-0,"647,25","-407,53125","-1761,34375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","34,1",
+2022-09-04 22:24:14,GCSpitfire,Oochorrs DN-F c13-0,"647,25","-407,53125","-1761,34375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","34,1",
+2022-09-04 22:24:31,CodexNecro81,Oochorrs PT-P d6-4,"696,625","-382,1875","-1758,03125",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","45,8",N
+2022-09-04 23:41:51,Arsinoe,Asterope,"-80,15625","-144,09375","-333,375",HR 1185,"-64,65625","-148,9375","-330,40625","16,5",
+2022-09-05 00:47:05,Gray Z7Z,Pleiades Sector PD-S b4-2,"-75,5625","-152,40625","-336,40625",Pleiades Sector GW-W c1-11,"-86,03125","-159,09375","-354,71875","22,1",
+2022-09-05 00:49:33,Gray Z7Z,Pleiades Sector GW-W c1-11,"-86,03125","-159,09375","-354,71875",Taygeta,"-88,5","-159,6875","-366,28125","11,8",
+2022-09-05 02:05:15,PanPiper,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-05 02:11:55,PanPiper,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-05 02:20:54,PanPiper,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",
+2022-09-05 02:29:05,Powell Oblivion,Oochorrs PT-P d6-0,667,"-418,15625","-1772,5",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","36,7",N
+2022-09-05 03:17:26,Rogixd,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",N
+2022-09-05 04:44:54,CP_Luiz,Synuefe GX-B c16-6,"-37,25","-55,125","-347,15625",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","10,6",Y
+2022-09-05 04:52:23,Seph Iridan,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625","10,5",Y
+2022-09-05 05:31:31,Rogixd,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625","10,5",Y
+2022-09-05 05:37:18,BL1P,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",N
+2022-09-05 05:41:34,Helmut Grokenberger,Oochorrs PT-P d6-0,667,"-418,15625","-1772,5",Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625","39,2",
+2022-09-05 05:44:24,PanPiper,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-05 05:46:32,PanPiper,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-05 05:48:18,LilacLight,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625","10,5",Y
+2022-09-05 05:53:46,SciberWolf,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-05 05:55:13,Rogixd,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",N
+2022-09-05 06:09:21,Rogixd,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",N
+2022-09-05 06:13:16,SciberWolf,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-05 06:14:37,SciberWolf,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-05 06:27:09,SciberWolf,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",
+2022-09-05 06:37:37,Me3se3ks,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625","10,5",Y
+2022-09-05 06:49:38,Me3se3ks,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",N
+2022-09-05 06:53:22,Rogixd,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",N
+2022-09-05 06:59:09,Me3se3ks,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",N
+2022-09-05 07:01:43,CP_Luiz,Witch Head Sector GR-W d1-9,"331,625","-348,65625","-653,4375",Witch Head Sector DL-Y d16,"350,5625","-369,46875","-701,5","55,7",N
+2022-09-05 07:10:25,Rogixd,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",N
+2022-09-05 07:22:23,Rogixd,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",N
+2022-09-05 07:31:47,Me3se3ks,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625","10,5",Y
+2022-09-05 07:41:54,Me3se3ks,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",N
+2022-09-05 07:51:16,CP_Luiz,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",N
+2022-09-05 08:04:39,CP_Luiz,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","57,2",N
+2022-09-05 08:09:06,Rogixd,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",N
+2022-09-05 08:13:41,CP_Luiz,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",N
+2022-09-05 08:19:46,CP_Luiz,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",N
+2022-09-05 08:26:28,CP_Luiz,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625","10,5",Y
+2022-09-05 08:30:53,CP_Luiz,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",N
+2022-09-05 08:37:49,CP_Luiz,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",N
+2022-09-05 08:42:14,Wotherspoon,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625","10,5",Y
+2022-09-05 08:49:27,CP_Luiz,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625","10,5",Y
+2022-09-05 09:11:30,CP_Luiz,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",N
+2022-09-05 09:30:29,Lou Snockers,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625","10,5",
+2022-09-05 09:46:22,Gaesatae,Pleiades Sector HR-W d1-57,"-86,40625","-125,15625","-301,84375",Celaeno,"-81,09375","-148,3125","-337,09375","42,5",N
+2022-09-05 10:04:24,Rogixd,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",N
+2022-09-05 10:39:26,Gaesatae,Pleione,-77,"-146,78125","-344,125",Pleiades Sector DL-Y d65,"-83,28125","-151,40625","-347,1875","8,4",N
+2022-09-05 10:54:17,Gaesatae,Pleiades Sector DL-Y d65,"-83,28125","-151,40625","-347,1875",Pleiades Sector PD-S b4-0,"-80,625","-156,6875","-337,4375","11,4",N
+2022-09-05 11:16:41,TomCatT,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector RO-Q b5-3,"-70,46875","-131,8125","-306,78125","30,9",
+2022-09-05 11:17:03,Lou Snockers,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",N
+2022-09-05 11:17:45,Rogixd,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625","10,5",Y
+2022-09-05 11:19:13,TomCatT,Pleiades Sector RO-Q b5-3,"-70,46875","-131,8125","-306,78125",Pleiades Sector YF-N b7-3,"-60,96875","-123,6875","-277,1875","32,1",
+2022-09-05 11:20:01,TomCatT,Pleiades Sector RO-Q b5-3,"-70,46875","-131,8125","-306,78125",Pleiades Sector YF-N b7-3,"-60,96875","-123,6875","-277,1875","32,1",
+2022-09-05 11:34:54,EREBUS5,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",N
+2022-09-05 11:49:47,Gaesatae,Celaeno,"-81,09375","-148,3125","-337,09375",Maia,"-81,78125","-149,4375","-343,375","6,4",N
+2022-09-05 11:57:24,Marko S Ramius,Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125",Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625","10,5",Y
+2022-09-05 12:08:01,ShoggothPete,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","10,5",N
+2022-09-05 12:13:19,Lou Snockers,Oochorrs YL-H c12-0,"658,875","-348,03125","-1806,15625",Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625","46,2",N
+2022-09-05 13:11:53,Heather G,Oochorrs DN-F c13-0,"647,25","-407,53125","-1761,34375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","34,1",
+2022-09-05 13:14:59,Heather G,Oochorrs DN-F c13-0,"647,25","-407,53125","-1761,34375",Oochorrs CS-F c13-0,"658,625","-384,21875","-1783,53125","34,1",
+2022-09-05 13:42:39,LakomLacen,Oochorrs UF-J c11-0,"686,125","-372,875","-1832,375",Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625","66,6",N
+2022-09-05 14:05:01,Notlike21,Synuefe GX-B c16-5,"-32,9375","-57,5625","-348,15625",HIP 22460,"-41,3125","-58,96875","-354,78125","10,8",Y
+2022-09-05 14:18:40,Maxwell Hauser [GPL],Pleiades Sector RY-R b4-0,"-76,3125","-171,3125","-335,0625",HIP 17862,"-81,4375","-151,90625","-359,59375","31,7",
+2022-09-05 15:02:33,LilacLight,Oochorrs ED-E c14-0,"658,1875","-343,28125","-1739,25",HD 38291,"619,25","-358,375",-1721,"45,6",N
+2022-09-05 15:27:27,Helmut Grokenberger,Oochorrs MY-P d6-6,"629,53125","-340,5","-1776,125",HD 38291,"619,25","-358,375",-1721,"58,9",N
+2022-09-05 15:39:36,Eckee,HD 38291,"619,25","-358,375",-1721,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","5,4",
+2022-09-05 15:46:19,Acred,HD 38291,"619,25","-358,375",-1721,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","5,4",Y
+2022-09-05 15:46:26,Eckee,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875",HD 38291,"619,25","-358,375",-1721,"5,4",
+2022-09-05 15:46:29,LilacLight,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875",HD 38291,"619,25","-358,375",-1721,"5,4",N
+2022-09-05 15:47:21,Eckee,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875",HD 38291,"619,25","-358,375",-1721,"5,4",
+2022-09-05 15:47:56,Marxanthius,Oochorrs ED-E c14-0,"658,1875","-343,28125","-1739,25",Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","42,3",N
+2022-09-05 15:49:11,Squeesquee,Taurus Dark Region VB-Z a15-0,"-53,46875","-42,40625","-373,625",HIP 22410,"-49,46875","-55,25","-360,59375","18,7",N
+2022-09-05 15:49:23,LilacLight,HD 38291,"619,25","-358,375",-1721,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","5,4",N
+2022-09-05 15:55:38,Eckee,Oochorrs PT-P d6-2,"666,3125","-353,9375","-1733,90625",Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","45,4",
+2022-09-05 15:58:13,TheProRockPL,HD 38291,"619,25","-358,375",-1721,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","5,4",N
+2022-09-05 16:03:22,Solace39,Pleiades Sector XF-N b7-1,"-77,09375",-121,"-278,65625",Pleiades Sector HR-W d1-79,"-80,625","-146,65625","-343,25","69,6",N
+2022-09-05 16:10:36,Acred,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875",HD 38291,"619,25","-358,375",-1721,"5,4",N
+2022-09-05 16:18:32,Acred,HD 38291,"619,25","-358,375",-1721,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","5,4",Y
+2022-09-05 16:21:20,Mass1nhibited,California Sector TT-R b4-0,"-331,09375","-247,0625","-913,125",California Sector LC-V c2-4,"-318,34375","-230,75","-888,21875","32,4",N
+2022-09-05 16:22:35,EREBUS5,Oochorrs DD-E c14-0,"636,09375","-327,96875","-1721,125",HD 38291,"619,25","-358,375",-1721,"34,8",N
+2022-09-05 16:22:52,BL1P,HD 38291,"619,25","-358,375",-1721,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","5,4",Y
+2022-09-05 16:25:47,Space_Trash,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",HD 38291,"619,25","-358,375",-1721,"68,7",
+2022-09-05 16:29:38,wizardkitty,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625",HD 38291,"619,25","-358,375",-1721,"26,8",N
+2022-09-05 16:30:34,Acred,HD 38291,"619,25","-358,375",-1721,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","5,4",Y
+2022-09-05 16:38:41,TheProRockPL,Oochorrs ED-E c14-0,"658,1875","-343,28125","-1739,25",Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","42,3",N
+2022-09-05 16:54:53,Marxanthius,Oochorrs OT-P d6-3,"606,625","-401,46875","-1736,5",Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","45,5",N
+2022-09-05 17:22:42,Radiumio,Oochorrs NK-A c16-0,"617,15625","-380,5625","-1662,1875",HD 38291,"619,25","-358,375",-1721,"62,9",N
+2022-09-05 17:24:43,Zantetzou,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",HD 38291,"619,25","-358,375",-1721,"68,7",N
+2022-09-05 17:35:15,Squeesquee,Taurus Dark Region VB-Z a15-0,"-53,46875","-42,40625","-373,625",HIP 22410,"-49,46875","-55,25","-360,59375","18,7",N
+2022-09-05 17:35:25,Zantetzou,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",HD 38291,"619,25","-358,375",-1721,"68,7",N
+2022-09-05 17:58:50,Firedrax,HIP 18544,"-53,21875","-159,625","-360,4375",Pleiades Sector HW-W c1-6,"-60,15625","-159,46875","-359,375",7,N
+2022-09-05 18:24:51,CP_Luiz,Witch Head Sector EB-X c1-8,"367,375","-379,1875","-734,9375",Shenve,"351,96875","-373,46875","-711,09375",29,N
+2022-09-05 18:25:43,Stukjes,Oochorrs HJ-C c15-0,"617,46875","-337,4375","-1683,625",HD 38291,"619,25","-358,375",-1721,"42,9",N
+2022-09-05 18:26:05,PUNKBUSTERS,Oochorrs SZ-N d7-1,"575,5625","-373,375","-1699,78125",HD 38291,"619,25","-358,375",-1721,"50,8",N
+2022-09-05 19:15:25,Alucardd,Shenve,"351,96875","-373,46875","-711,09375",Witch Head Sector TJ-Q b5-0,"359,71875","-404,1875","-701,96875",33,N
+2022-09-05 19:23:23,HunabKU,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875",HD 38291,"619,25","-358,375",-1721,"5,4",N
+2022-09-05 19:24:09,BL1P,HD 38291,"619,25","-358,375",-1721,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","5,4",Y
+2022-09-05 19:43:55,badloop,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","63,4",
+2022-09-05 19:46:49,IvIePhisto,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","63,4",
+2022-09-05 19:48:35,CP_Luiz,Taurus Dark Region AQ-O b6-1,"-1,46875","-101,96875","-400,875",Pleiades Sector FR-U b3-0,"-38,4375",-69,"-359,96875","64,2",N
+2022-09-05 19:49:26,00rt,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625",HD 38291,"619,25","-358,375",-1721,"26,8",
+2022-09-05 20:30:17,Argonaut Proxi,HD 38291,"619,25","-358,375",-1721,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","5,4",Y
+2022-09-05 20:33:49,GraphicEqualizer,Pleiades Sector DL-X b1-1,"-72,875","-160,84375","-387,6875",Merope,"-78,59375","-149,625","-340,53125","48,8",N
+2022-09-05 21:03:42,BL1P,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625",HD 38291,"619,25","-358,375",-1721,"26,8",N
+2022-09-05 21:22:11,00rt,HD 38291,"619,25","-358,375",-1721,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","5,4",
+2022-09-05 21:23:58,BL1P,HD 38291,"619,25","-358,375",-1721,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","5,4",Y
+2022-09-05 21:35:04,KYLAE,Oochorrs DD-E c14-0,"636,09375","-327,96875","-1721,125",HD 38291,"619,25","-358,375",-1721,"34,8",N
+2022-09-05 21:38:20,LilacLight,Oochorrs ED-E c14-0,"658,1875","-343,28125","-1739,25",HD 38291,"619,25","-358,375",-1721,"45,6",N
+2022-09-05 21:56:35,Mr.DiGi,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875",HD 38291,"619,25","-358,375",-1721,"5,4",
+2022-09-05 22:02:56,ZEN Industries,Pleiades Sector WP-O b6-0,"-104,1875","-162,21875","-303,96875",Pleiades Sector HR-W d1-35,"-87,21875","-158,875","-281,96875",28,N
+2022-09-05 22:14:08,Cpt Favia,Taygeta,"-88,5","-159,6875","-366,28125",Electra,"-86,0625","-159,9375","-361,65625","5,2",
+2022-09-05 22:31:26,Cpt Favia,Electra,"-86,0625","-159,9375","-361,65625",Taygeta,"-88,5","-159,6875","-366,28125","5,2",
+2022-09-05 23:26:16,Alfredo Cavatelli,Oochorrs QE-O d7-0,"580,1875","-343,71875","-1695,375",HD 38291,"619,25","-358,375",-1721,49,N
+2022-09-05 23:34:10,CodexNecro81,Oochorrs ED-E c14-0,"658,1875","-343,28125","-1739,25",HD 38291,"619,25","-358,375",-1721,"45,6",N
+2022-09-05 23:38:22,CodexNecro81,HD 38291,"619,25","-358,375",-1721,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","5,4",Y
+2022-09-05 23:51:26,CP_Luiz,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",HD 38291,"619,25","-358,375",-1721,"68,7",N
+2022-09-06 00:12:52,CP_Luiz,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",HD 38291,"619,25","-358,375",-1721,"68,7",N
+2022-09-06 00:21:07,CP_Luiz,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",HD 38291,"619,25","-358,375",-1721,"68,7",N
+2022-09-06 00:47:33,CP_Luiz,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875",HD 38291,"619,25","-358,375",-1721,"5,4",N
+2022-09-06 00:53:55,Billy Shakes,HIP 17552,"-51,59375","-126,21875","-262,125",Pleiades Sector KS-T c3-14,"-67,65625","-103,59375","-290,90625",40,N
+2022-09-06 01:23:01,Gray Z7Z,Taygeta,"-88,5","-159,6875","-366,28125",HR 1172,"-75,1875","-152,40625","-346,59375","24,9",N
+2022-09-06 01:29:13,Rogixd,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",HD 38291,"619,25","-358,375",-1721,"68,7",N
+2022-09-06 01:44:28,Gray Z7Z,HR 1172,"-75,1875","-152,40625","-346,59375",Maia,"-81,78125","-149,4375","-343,375","7,9",N
+2022-09-06 02:00:41,Denis Page,HIP 22918,"-3,25","-93,28125","-424,1875",Taurus Dark Region YF-N b7-1,"-39,84375","-57,34375","-378,1875","68,9",
+2022-09-06 02:06:34,Gaia_origin,Oochorrs CD-E c14-1,"609,8125","-322,53125","-1739,03125",HD 38291,"619,25","-358,375",-1721,"41,2",N
+2022-09-06 02:07:59,Denis Page,HIP 22918,"-3,25","-93,28125","-424,1875",Taurus Dark Region YF-N b7-1,"-39,84375","-57,34375","-378,1875","68,9",
+2022-09-06 02:09:38,Denis Page,Taurus Dark Region UZ-O b6-1,"-32,96875","-59,34375","-385,53125",Taurus Dark Region XF-N b7-1,"-49,78125","-48,625","-366,4375","27,6",
+2022-09-06 02:36:41,pSyren Farseer,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","63,4",N
+2022-09-06 02:57:18,CP_Luiz,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625",Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","29,3",N
+2022-09-06 03:09:28,CP_Luiz,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625",Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","29,3",N
+2022-09-06 03:36:08,PUNKBUSTERS,HD 38291,"619,25","-358,375",-1721,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","5,4",N
+2022-09-06 03:36:43,CP_Luiz,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625",Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","29,3",N
+2022-09-06 04:07:56,CP_Luiz,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625",Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","29,3",N
+2022-09-06 05:08:52,CP_Luiz,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875",HD 38291,"619,25","-358,375",-1721,"5,4",N
+2022-09-06 05:49:01,67MistakeNot,Oochorrs MY-P d6-5,"616,5","-336,75","-1761,6875",HD 38291,"619,25","-358,375",-1721,"46,2",
+2022-09-06 05:56:45,BL1P,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625",HD 38291,"619,25","-358,375",-1721,"26,8",N
+2022-09-06 06:17:15,CP_Luiz,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",HD 38291,"619,25","-358,375",-1721,"68,7",N
+2022-09-06 06:33:06,Stivl Writh,HIP 20933,"-58,125","-97,71875","-392,09375",Pleiades Sector CQ-Y d60,"-46,21875","-83,5",-367,"31,2",N
+2022-09-06 09:14:52,Powell Oblivion,Oochorrs DD-E c14-0,"636,09375","-327,96875","-1721,125",HD 38291,"619,25","-358,375",-1721,"34,8",N
+2022-09-06 09:30:47,BL1P,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",HD 38291,"619,25","-358,375",-1721,"68,7",N
+2022-09-06 09:37:39,BL1P,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625",HD 38291,"619,25","-358,375",-1721,"26,8",N
+2022-09-06 09:45:13,BL1P,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625",HD 38291,"619,25","-358,375",-1721,"26,8",N
+2022-09-06 10:05:09,Xynima,Oochorrs QE-O d7-0,"580,1875","-343,71875","-1695,375",Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","54,1",N
+2022-09-06 10:15:20,Rogixd,Oochorrs DN-F c13-0,"647,25","-407,53125","-1761,34375",HD 38291,"619,25","-358,375",-1721,"69,5",N
+2022-09-06 10:17:24,Xynima,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875",HD 38291,"619,25","-358,375",-1721,"5,4",N
+2022-09-06 11:29:30,Rogixd,Oochorrs DN-F c13-0,"647,25","-407,53125","-1761,34375",HD 38291,"619,25","-358,375",-1721,"69,5",N
+2022-09-06 11:33:14,LilacLight,Oochorrs IE-C c15-0,"580,40625","-381,125","-1703,28125",HD 38291,"619,25","-358,375",-1721,"48,4",N
+2022-09-06 11:38:43,Rogixd,Oochorrs DN-F c13-0,"647,25","-407,53125","-1761,34375",HD 38291,"619,25","-358,375",-1721,"69,5",N
+2022-09-06 12:13:10,Rogixd,Oochorrs DN-F c13-0,"647,25","-407,53125","-1761,34375",HD 38291,"619,25","-358,375",-1721,"69,5",N
+2022-09-06 12:53:46,Rogixd,Oochorrs DN-F c13-0,"647,25","-407,53125","-1761,34375",HD 38291,"619,25","-358,375",-1721,"69,5",N
+2022-09-06 13:07:38,Rogixd,Oochorrs DN-F c13-0,"647,25","-407,53125","-1761,34375",HD 38291,"619,25","-358,375",-1721,"69,5",N
+2022-09-06 13:09:11,LilacLight,Oochorrs OT-P d6-3,"606,625","-401,46875","-1736,5",HD 38291,"619,25","-358,375",-1721,"47,5",N
+2022-09-06 13:12:55,T'Verez,Oochorrs PT-P d6-1,"666,3125","-350,5625","-1720,09375",Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","45,4",
+2022-09-06 13:28:17,Mallchad,Witch Head Sector XE-Q b5-0,"396,4375","-424,15625","-699,875",Witch Head Sector NX-U c2-6,"388,5","-426,34375","-694,65625","9,7",
+2022-09-06 13:43:41,Alucardd,Pleiades Sector UO-Q b5-3,"-20,78125","-144,125","-323,59375",Pleiades Sector SO-Q b5-0,"-46,59375","-129,53125","-309,8125","32,7",N
+2022-09-06 13:45:07,Fallharbor,Taygeta,"-88,5","-159,6875","-366,28125",Electra,"-86,0625","-159,9375","-361,65625","5,2",N
+2022-09-06 13:45:51,LilacLight,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875",HD 38291,"619,25","-358,375",-1721,"5,4",N
+2022-09-06 13:56:21,LilacLight,HD 38291,"619,25","-358,375",-1721,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","5,4",Y
+2022-09-06 14:02:19,LilacLight,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875",HD 38291,"619,25","-358,375",-1721,"5,4",N
+2022-09-06 14:09:13,LilacLight,HD 38291,"619,25","-358,375",-1721,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","5,4",Y
+2022-09-06 14:20:07,Rogixd,Oochorrs DN-F c13-0,"647,25","-407,53125","-1761,34375",HD 38291,"619,25","-358,375",-1721,"69,5",N
+2022-09-06 14:26:28,LilacLight,HD 38291,"619,25","-358,375",-1721,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","5,4",Y
+2022-09-06 14:48:35,Powell Oblivion,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625",HD 38291,"619,25","-358,375",-1721,"26,8",N
+2022-09-06 14:52:09,Powell Oblivion,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625",HD 38291,"619,25","-358,375",-1721,"26,8",N
+2022-09-06 14:54:39,Rogixd,Oochorrs DN-F c13-0,"647,25","-407,53125","-1761,34375",HD 38291,"619,25","-358,375",-1721,"69,5",N
+2022-09-06 15:14:01,Powell Oblivion,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625",HD 38291,"619,25","-358,375",-1721,"26,8",N
+2022-09-06 15:17:16,Foperider,Pleiades Sector CQ-Y d58,"-53,5625","-60,9375","-353,03125",Pleiades Sector FR-V c2-1,"-58,125","-60,59375","-343,9375","10,2",Y
+2022-09-06 15:31:49,Rogixd,HD 38291,"619,25","-358,375",-1721,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","5,4",Y
+2022-09-06 16:14:06,Rogixd,Oochorrs PT-P d6-2,"666,3125","-353,9375","-1733,90625",HD 38291,"619,25","-358,375",-1721,49,N
+2022-09-06 16:29:47,M. V. Coehoorn,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","63,4",
+2022-09-06 16:31:00,M. V. Coehoorn,Oochorrs BS-F c13-0,"650,46875","-382,9375","-1777,0625",Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","63,4",
+2022-09-06 16:40:35,Rogixd,HD 38291,"619,25","-358,375",-1721,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","5,4",Y
+2022-09-06 17:06:36,Squeesquee,HIP 22460,"-41,3125","-58,96875","-354,78125",Taurus Dark Region XF-N b7-1,"-49,78125","-48,625","-366,4375","17,7",N
+2022-09-06 17:21:28,Iku Turso,Oochorrs NK-A c16-0,"617,15625","-380,5625","-1662,1875",HD 38291,"619,25","-358,375",-1721,"62,9",
+2022-09-06 17:37:36,LilacLight,Oochorrs MY-P d6-3,"588,6875","-322,40625","-1733,25",HD 38291,"619,25","-358,375",-1721,"48,8",N
+2022-09-06 19:02:09,LilacLight,Oochorrs ED-E c14-0,"658,1875","-343,28125","-1739,25",HD 38291,"619,25","-358,375",-1721,"45,6",N
+2022-09-06 19:11:03,Slipi,Pleiades Sector IG-X b1-1,"-14,1875","-178,46875","-402,0625",Pleiades Sector KH-V c2-14,"-22,84375","-143,40625","-330,84375","79,9",
+2022-09-06 19:30:59,teeter jenkins,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625",HD 38291,"619,25","-358,375",-1721,"26,8",N
+2022-09-06 19:42:22,teeter jenkins,Oochorrs CD-E c14-1,"609,8125","-322,53125","-1739,03125",HD 38291,"619,25","-358,375",-1721,"41,2",N
+2022-09-06 19:48:05,teeter jenkins,Oochorrs MY-P d6-4,"589,6875","-330,5625","-1724,15625",HD 38291,"619,25","-358,375",-1721,"40,7",N
+2022-09-06 20:15:03,teeter jenkins,Oochorrs DD-E c14-0,"636,09375","-327,96875","-1721,125",HD 38291,"619,25","-358,375",-1721,"34,8",N
+2022-09-06 20:24:47,LilacLight,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875",HD 38291,"619,25","-358,375",-1721,"5,4",N
+2022-09-06 21:02:12,Sindri Völuspa,Musca Dark Region HM-V c2-29,"418,46875","-4,5625","270,65625",HIP 62154,"411,375","9,09375","261,46875","17,9",N
+2022-09-06 21:34:36,pokegustavo,Taurus Dark Region ZF-N b7-1,"-23,34375","-51,28125","-373,875",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","32,5",
+2022-09-06 21:46:13,BL1P,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625",HD 38291,"619,25","-358,375",-1721,"26,8",N
+2022-09-06 21:59:09,Mallchad,Oochorrs EY-D c14-0,"577,375","-380,6875",-1741,HD 38291,"619,25","-358,375",-1721,"51,5",
+2022-09-06 22:00:49,Mallchad,Oochorrs EY-D c14-0,"577,375","-380,6875",-1741,HD 38291,"619,25","-358,375",-1721,"51,5",
+2022-09-06 22:02:50,CodexNecro81,Oochorrs OT-P d6-3,"606,625","-401,46875","-1736,5",HD 38291,"619,25","-358,375",-1721,"47,5",N
+2022-09-06 22:09:15,Helmut Grokenberger,HD 38291,"619,25","-358,375",-1721,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","5,4",Y
+2022-09-06 22:19:05,Lou Snockers,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875",HD 38291,"619,25","-358,375",-1721,"5,4",N
+2022-09-06 22:24:20,pokegustavo,Col 285 Sector GL-X c1-11,"-42,3125","-65,65625","-302,3125",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","49,5",
+2022-09-06 22:30:06,BL1P,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625",Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","29,3",N
+2022-09-06 22:47:24,pokegustavo,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",Col 285 Sector GL-X c1-11,"-42,3125","-65,65625","-302,3125","49,5",
+2022-09-06 22:59:54,Eckee,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625",HD 38291,"619,25","-358,375",-1721,"26,8",N
+2022-09-06 23:02:18,BL1P,Oochorrs PT-P d6-1,"666,3125","-350,5625","-1720,09375",Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875","45,4",N
+2022-09-06 23:09:51,Eckee,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625",HD 38291,"619,25","-358,375",-1721,"26,8",N
+2022-09-06 23:30:10,SIX-SHOOTER,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875",HD 38291,"619,25","-358,375",-1721,"5,4",
+2022-09-06 23:48:03,pokegustavo,Pleiades Sector GW-W d1-33,"-50,25","-62,8125","-326,8125",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","25,4",
+2022-09-06 23:59:57,Mallchad,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875",HD 38291,"619,25","-358,375",-1721,"5,4",
+2022-09-07 00:34:07,pokegustavo,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",
+2022-09-07 04:56:03,ProCactus167,Asterope,"-80,15625","-144,09375","-333,375",Celaeno,"-81,09375","-148,3125","-337,09375","5,7",N
+2022-09-07 07:35:05,CP_Luiz,Oochorrs PT-P d6-2,"666,3125","-353,9375","-1733,90625",HD 38291,"619,25","-358,375",-1721,49,N
+2022-09-07 07:41:42,CP_Luiz,Oochorrs PT-P d6-2,"666,3125","-353,9375","-1733,90625",HD 38291,"619,25","-358,375",-1721,49,N
+2022-09-07 08:48:45,Helmut Grokenberger,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875",HD 38291,"619,25","-358,375",-1721,"5,4",N
+2022-09-07 09:30:45,CP_Luiz,Oochorrs PT-P d6-2,"666,3125","-353,9375","-1733,90625",HD 38291,"619,25","-358,375",-1721,49,N
+2022-09-07 13:12:34,LilacLight,Oochorrs SZ-N d7-4,"637,5",-349,"-1676,53125",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","24,1",N
+2022-09-07 13:26:06,Eckee,Oochorrs HJ-C c15-0,"617,46875","-337,4375","-1683,625",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,8",N
+2022-09-07 14:21:58,LilacLight,Oochorrs IJ-C c15-0,"658,1875","-340,8125","-1699,1875",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","25,6",N
+2022-09-07 15:02:51,HunabKU,HD 38291,"619,25","-358,375",-1721,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,8",N
+2022-09-07 15:47:30,Squeesquee,HIP 22410,"-49,46875","-55,25","-360,59375",HIP 22460,"-41,3125","-58,96875","-354,78125","10,7",N
+2022-09-07 17:04:18,Käptn Peng,Pleiades Sector EB-X c1-3,"-65,125","-109,875","-358,46875",Pleiades Sector DG-X c1-10,"-53,34375","-66,8125","-357,28125","44,7",N
+2022-09-07 17:09:01,Manu Wörkl,Taurus Dark Region YF-N b7-4,"-29,4375","-61,96875","-367,9375",HR 1554,"-32,375","-57,53125","-340,65625","27,8",Y
+2022-09-07 17:12:07,Käptn Peng,Pleiades Sector DG-X c1-10,"-53,34375","-66,8125","-357,28125",HIP 22460,"-41,3125","-58,96875","-354,78125","14,6",N
+2022-09-07 17:37:31,TheProRockPL,HIP 17892,"-70,75","-157,9375","-352,84375",Maia,"-81,78125","-149,4375","-343,375","16,8",N
+2022-09-07 17:52:48,Gaesatae,Celaeno,"-81,09375","-148,3125","-337,09375",Pleiades Sector JR-V b2-1,"-33,0625","-163,90625","-379,28125","65,8",N
+2022-09-07 19:42:41,badloop,HD 38291,"619,25","-358,375",-1721,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,8",N
+2022-09-07 19:52:07,PanPiper,Oochorrs IC-U e3-1,"602,90625","-344,5","-1656,46875",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","54,2",N
+2022-09-07 19:56:42,Molan Ryke,Oochorrs IC-U e3-1,"602,90625","-344,5","-1656,46875",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","54,2",N
+2022-09-07 20:08:05,Marko S Ramius,HD 38291,"619,25","-358,375",-1721,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,8",N
+2022-09-07 20:20:22,PeterBob,Oochorrs TZ-N d7-2,"689,59375","-365,3125","-1674,21875",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","63,1",N
+2022-09-07 20:45:30,Comlag1984,HD 38291,"619,25","-358,375",-1721,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,8",N
+2022-09-07 21:38:55,Slipi,Pleiades Sector BL-W b2-0,"-35,78125","-67,21875","-366,90625",HIP 22460,"-41,3125","-58,96875","-354,78125","15,7",Y
+2022-09-07 21:49:57,Slipi,Pleiades Sector BV-Y c1,"-45,0625","-106,78125","-405,375",Pleiades Sector XO-Z b0,-41,"-124,375","-421,125",24,N
+2022-09-07 22:11:16,pokegustavo,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","5,2",
+2022-09-07 22:45:06,GCSpitfire,HD 38291,"619,25","-358,375",-1721,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,8",N
+2022-09-07 22:51:31,nyzchf,Witch Head Sector CF-Z b0,"417,8125","-399,40625","-802,3125",Witch Head Sector KM-V b2-0,"375,78125","-422,875","-761,75",63,N
+2022-09-07 23:50:46,BlakeA,Taygeta,"-88,5","-159,6875","-366,28125",Pleiades Sector KC-V c2-16,"-81,71875","-155,5","-340,84375","26,7",
+2022-09-08 00:31:03,CP_Luiz,Taurus Dark Region BB-N b7-1,"-22,4375","-65,34375","-366,28125",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","27,1",Y
+2022-09-08 00:31:31,Qianas T. Geeq,HD 38291,"619,25","-358,375",-1721,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,8",N
+2022-09-08 00:55:01,Mallchad,Oochorrs RE-O d7-2,"668,125","-320,8125","-1673,75",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625",52,N
+2022-09-08 01:11:18,pokegustavo,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","29,3",
+2022-09-08 01:24:02,Novaseta,HD 38291,"619,25","-358,375",-1721,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,8",
+2022-09-08 02:29:16,Darth_Vader,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",Pleiades Sector CQ-Y d58,"-53,5625","-60,9375","-353,03125","8,9",
+2022-09-08 02:31:38,Darth_Vader,Pleiades Sector CQ-Y d58,"-53,5625","-60,9375","-353,03125",Pleiades Sector FR-V c2-1,"-58,125","-60,59375","-343,9375","10,2",
+2022-09-08 02:33:31,CP_Luiz,Oochorrs IJ-C c15-0,"658,1875","-340,8125","-1699,1875",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","25,6",N
+2022-09-08 02:56:52,CP_Luiz,Oochorrs IJ-C c15-0,"658,1875","-340,8125","-1699,1875",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","25,6",N
+2022-09-08 03:26:42,Marzyx,Pleiades Sector GW-W d1-33,"-50,25","-62,8125","-326,8125",HIP 22460,"-41,3125","-58,96875","-354,78125","29,6",
+2022-09-08 03:53:40,CP_Luiz,Oochorrs PT-P d6-1,"666,3125","-350,5625","-1720,09375",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","37,6",N
+2022-09-08 04:01:06,CP_Luiz,Oochorrs PT-P d6-1,"666,3125","-350,5625","-1720,09375",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","37,6",N
+2022-09-08 04:02:31,Historia-g,HIP 19478,"27,40625","-218,03125","-383,46875",Merope,"-78,59375","-149,625","-340,53125","133,3",N
+2022-09-08 04:38:27,Historia-g,HIP 17125,"-95,875","-128,75","-316,5625",HIP 17403,"-93,6875","-158,96875","-367,625","59,4",N
+2022-09-08 04:57:43,Historia-g,HIP 17403,"-93,6875","-158,96875","-367,625",Merope,"-78,59375","-149,625","-340,53125","32,4",N
+2022-09-08 05:04:30,Historia-g,Pleiades Sector HM-V c2-5,"-45,875","-93,53125","-344,78125",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","33,1",Y
+2022-09-08 05:23:07,CP_Luiz,Oochorrs PT-P d6-1,"666,3125","-350,5625","-1720,09375",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","37,6",N
+2022-09-08 05:33:59,CP_Luiz,Oochorrs PT-P d6-1,"666,3125","-350,5625","-1720,09375",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","37,6",N
+2022-09-08 05:34:07,crux br,HD 38291,"619,25","-358,375",-1721,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,8",N
+2022-09-08 06:21:50,van hoof,Synuefe RA-C b33-2,"-43,78125","-46,15625","-350,65625",Col 285 Sector BD-Z a2-0,"-31,1875","-43,34375","-319,59375","33,6",
+2022-09-08 10:17:37,psykit,Synuefe KD-A c17-10,"-32,375","-58,1875","-332,6875",Synuefe GX-B c16-6,"-37,25","-55,125","-347,15625","15,6",
+2022-09-08 10:19:43,psykit,Synuefe GX-B c16-6,"-37,25","-55,125","-347,15625",HIP 22460,"-41,3125","-58,96875","-354,78125","9,5",
+2022-09-08 10:44:06,Kaito Tatsuhiro,Oochorrs TZ-N d7-4,"682,6875","-357,25","-1676,34375",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","54,6",N
+2022-09-08 11:03:38,SAWAMURA_ERIRI,Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875",HIP 22460,"-41,3125","-58,96875","-354,78125","8,4",Y
+2022-09-08 11:22:16,psykit,HD 38291,"619,25","-358,375",-1721,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,8",
+2022-09-08 11:34:21,Powell Oblivion,Oochorrs PT-P d6-1,"666,3125","-350,5625","-1720,09375",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","37,6",N
+2022-09-08 11:34:21,SAWAMURA_ERIRI,Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875",HIP 22460,"-41,3125","-58,96875","-354,78125","8,4",Y
+2022-09-08 11:37:53,SAWAMURA_ERIRI,Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875",HIP 22460,"-41,3125","-58,96875","-354,78125","8,4",Y
+2022-09-08 12:07:59,Radiumio,Taurus Dark Region KH-V c2-13,"-5,8125","-77,65625","-387,71875",HIP 22460,"-41,3125","-58,96875","-354,78125","51,9",N
+2022-09-08 13:02:37,psykit,Delphi,"-63,59375","-147,40625","-319,09375",Pleiades Sector HM-V c2-5,"-45,875","-93,53125","-344,78125","62,3",
+2022-09-08 13:15:34,T'Verez,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","29,3",
+2022-09-08 13:16:45,T'Verez,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","29,3",
+2022-09-08 13:41:09,Luriant,Pleiades Sector DB-X c1-9,"-118,28125","-134,75",-360,Pleiades Sector DL-Y d62,"-92,21875","-107,59375","-358,75","37,7",N
+2022-09-08 13:46:06,Luriant,Pleiades Sector DR-U b3-3,"-69,71875","-76,84375","-356,71875",HIP 22460,"-41,3125","-58,96875","-354,78125","33,6",Y
+2022-09-08 14:11:46,Mallchad,HD 38291,"619,25","-358,375",-1721,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,8",N
+2022-09-08 14:33:30,Mass1nhibited,California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375",HIP 18390,"-299,0625","-229,25","-876,125","22,2",N
+2022-09-08 15:02:28,REFUNDIAN,Merope,"-78,59375","-149,625","-340,53125",Pleiades Sector AB-N b7-0,"-54,1875","-140,40625","-269,75","75,4",N
+2022-09-08 15:05:18,M. V. Coehoorn,Taygeta,"-88,5","-159,6875","-366,28125",Delphi,"-63,59375","-147,40625","-319,09375","54,8",
+2022-09-08 15:22:17,Mallchad,HD 38291,"619,25","-358,375",-1721,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,8",N
+2022-09-08 15:25:51,Sayaka,Oochorrs LP-A c16-0,"616,1875","-341,9375","-1664,90625",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","40,6",N
+2022-09-08 16:01:55,Mallchad,HD 38291,"619,25","-358,375",-1721,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,8",N
+2022-09-08 16:21:04,Mass1nhibited,HIP 18390,"-299,0625","-229,25","-876,125",California Sector HR-W d1-25,"-318,9375","-223,40625","-904,96875","35,5",N
+2022-09-08 16:36:57,Fallharbor,Electra,"-86,0625","-159,9375","-361,65625",HIP 17225,"-86,15625","-165,59375","-356,8125","7,4",N
+2022-09-08 16:43:48,Gargantuan Jockey,Pleiades Sector WZ-X b1-4,-93,"-96,15625","-385,59375",Pleiades Sector HX-S b4-1,"-78,28125","-77,15625","-343,34375","48,6",N
+2022-09-08 17:20:35,Galactik,Oochorrs OT-P d6-3,"606,625","-401,46875","-1736,5",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","68,7",
+2022-09-08 17:38:14,Helmut Grokenberger,Oochorrs SZ-N d7-4,"637,5",-349,"-1676,53125",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","24,1",N
+2022-09-08 18:32:41,GCSpitfire,HD 38291,"619,25","-358,375",-1721,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,8",N
+2022-09-08 18:45:37,Fallharbor,HIP 16753,"-88,3125","-172,9375","-348,84375",Pleiades Sector GW-W c1-13,"-77,375","-156,3125","-352,03125","20,2",N
+2022-09-08 19:29:14,Gaesatae,Taurus Dark Region UT-R b4-2,"-27,8125","-129,1875","-439,34375",Pleiades Sector FB-X c1-3,"-43,4375","-140,96875","-373,96875","68,2",N
+2022-09-08 19:31:21,Gaesatae,Pleiades Sector FB-X c1-3,"-43,4375","-140,96875","-373,96875",Celaeno,"-81,09375","-148,3125","-337,09375","53,2",N
+2022-09-08 19:48:30,Fallharbor,Pleiades Sector HR-W d1-79,"-80,625","-146,65625","-343,25",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125","7,2",N
+2022-09-08 19:54:03,TheMightySardine,Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875",Asterope,"-80,15625","-144,09375","-333,375","22,8",N
+2022-09-08 20:21:40,Stefanos,Pleiades Sector IH-V c2-7,"-78,46875","-144,9375","-324,9375",Asterope,"-80,15625","-144,09375","-333,375","8,6",N
+2022-09-08 20:25:09,Stefanos,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector IH-V c2-7,"-78,46875","-144,9375","-324,9375","8,6",N
+2022-09-08 21:38:20,Deathcow,HD 38291,"619,25","-358,375",-1721,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,8",N
+2022-09-08 22:43:02,TheMightySardine,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875","22,8",N
+2022-09-08 22:46:26,Chewcat1,Oochorrs FY-D c14-0,"622,25","-360,34375","-1724,96875",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","29,3",N
+2022-09-08 23:03:53,Fallharbor,Pleiades Sector EB-X c1-3,"-65,125","-109,875","-358,46875",HIP 22460,"-41,3125","-58,96875","-354,78125","56,3",N
+2022-09-08 23:39:01,pokegustavo,Taurus Dark Region ON-T c3-10,"-12,0625","-74,9375","-380,65625",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","45,9",
+2022-09-08 23:46:53,Dark3982,Pleiades Sector IX-S b4-0,"-61,25","-84,25","-341,875",Pleiades Sector HC-T b4-0,"-43,25","-60,03125","-343,03125","30,2",Y
+2022-09-09 00:55:41,Historia-g,Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875",Pleiades Sector IH-V c2-0,"-104,3125","-140,4375","-344,78125","4,4",N
+2022-09-09 01:19:25,pokegustavo,Col 285 Sector CP-Y b1-3,"-45,96875","-55,75","-324,625",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","27,5",
+2022-09-09 01:26:42,CP_Luiz,HIP 22711,"-35,75","-60,84375","-366,90625",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","17,8",Y
+2022-09-09 01:54:37,CP_Luiz,HIP 22410,"-49,46875","-55,25","-360,59375",Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875","5,6",Y
+2022-09-09 01:58:33,CP_Luiz,Pleiades Sector CW-U b3-3,"-50,5625","-63,53125","-362,5",Pleiades Sector CW-U b3-4,"-54,5","-64,875","-359,9375","4,9",Y
+2022-09-09 02:03:19,CP_Luiz,Pleiades Sector CW-U b3-3,"-50,5625","-63,53125","-362,5",Pleiades Sector CW-U b3-4,"-54,5","-64,875","-359,9375","4,9",Y
+2022-09-09 02:07:00,CP_Luiz,Pleiades Sector CW-U b3-4,"-54,5","-64,875","-359,9375",Pleiades Sector DG-X c1-12,"-52,5","-70,6875","-365,75","8,5",Y
+2022-09-09 03:05:50,CP_Luiz,Pleiades Sector DG-X c1-15,"-55,09375","-101,65625","-353,375",HIP 22460,"-41,3125","-58,96875","-354,78125","44,9",Y
+2022-09-09 03:16:15,Arsinoe,Pleiades Sector JC-V d2-62,"-82,1875","-100,9375","-260,59375",Pleiades Sector HR-W d1-25,"-91,34375","-114,71875","-321,1875","62,8",N
+2022-09-09 03:27:39,tokisu,California Sector MX-T b3-0,"-312,4375","-211,9375","-932,59375",California Sector DL-Y d2,"-320,46875","-204,1875","-925,53125","13,2",N
+2022-09-09 03:44:39,tokisu,California Sector NI-S b4-0,"-344,3125","-201,34375","-923,25",California Sector LX-T b3-0,"-337,90625","-211,9375","-927,03125","12,9",N
+2022-09-09 04:40:28,tokisu,California Sector IR-W d1-23,"-302,53125","-228,5625","-888,0625",California Sector KH-V c2-0,-301,"-221,53125","-901,875","15,6",N
+2022-09-09 05:07:39,BL1P,Merope,"-78,59375","-149,625","-340,53125",Pleiades Sector KX-T b3-1,"-100,28125","-153,53125","-346,03125","22,7",N
+2022-09-09 05:30:13,BL1P,Pleiades Sector HR-W d1-78,"-93,0625","-151,65625","-340,1875",Merope,"-78,59375","-149,625","-340,53125","14,6",N
+2022-09-09 06:45:55,Novaseta,HD 38291,"619,25","-358,375",-1721,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,8",
+2022-09-09 08:17:52,Maxwell Hauser [GPL],HD 38291,"619,25","-358,375",-1721,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,8",N
+2022-09-09 11:51:03,LilacLight,Evangelis,"353,75","-419,03125",-710,Witch Head Sector DL-Y d8,"351,75","-419,65625","-706,5","4,1",N
+2022-09-09 12:30:46,Louis Calvert,Oochorrs SZ-N d7-4,"637,5",-349,"-1676,53125",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","24,1",N
+2022-09-09 12:38:37,LilacLight,Oochorrs HJ-C c15-0,"617,46875","-337,4375","-1683,625",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,8",N
+2022-09-09 13:23:42,Mallchad,Oochorrs SZ-N d7-5,"642,625","-345,5","-1676,125",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,1",N
+2022-09-09 13:34:20,Marxanthius,Oochorrs DD-E c14-0,"636,09375","-327,96875","-1721,125",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","30,3",N
+2022-09-09 13:34:57,Marko S Ramius,HD 38291,"619,25","-358,375",-1721,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,8",N
+2022-09-09 13:42:02,Neutronic1,HD 38291,"619,25","-358,375",-1721,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,8",N
+2022-09-09 14:00:14,Henoch,Oochorrs SZ-N d7-4,"637,5",-349,"-1676,53125",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","24,1",N
+2022-09-09 14:02:49,Stukjes,Oochorrs SZ-N d7-5,"642,625","-345,5","-1676,125",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,1",N
+2022-09-09 14:11:57,Stukjes,Oochorrs SZ-N d7-5,"642,625","-345,5","-1676,125",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,1",N
+2022-09-09 14:17:51,Neutronic1,HD 38291,"619,25","-358,375",-1721,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,8",N
+2022-09-09 14:48:05,zmouliche,Pleiades Sector GM-U b3-3,"-49,875","-101,09375","-358,71875",HIP 22460,"-41,3125","-58,96875","-354,78125","43,2",N
+2022-09-09 14:49:23,BL1P,HIP 22410,"-49,46875","-55,25","-360,59375",Synuefe GX-B c16-5,"-32,9375","-57,5625","-348,15625","20,8",Y
+2022-09-09 14:56:10,zmouliche,Pleiades Sector GM-U b3-1,-51,"-101,84375","-360,90625",HIP 22460,"-41,3125","-58,96875","-354,78125","44,4",N
+2022-09-09 15:13:21,zmouliche,Pleiades Sector PO-Q b5-0,"-121,6875","-141,46875","-324,75",HIP 16171,"-107,875","-155,6875","-331,65625",21,N
+2022-09-09 15:49:58,Marxanthius,Slegi GS-X b45-0,"-2016,65625","-654,6875","-2637,65625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","25,1",N
+2022-09-09 15:50:35,LilacLight,Slegi GS-X b45-0,"-2016,65625","-654,6875","-2637,65625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","25,1",N
+2022-09-09 15:51:33,BL1P,Pleiades Sector CW-U b3-1,"-46,53125","-62,9375","-364,5625",Pleiades Sector HC-T b4-0,"-43,25","-60,03125","-343,03125",22,Y
+2022-09-09 15:59:27,LilacLight,Slegi FX-X b45-0,"-2000,53125","-643,4375","-2639,125",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","14,8",N
+2022-09-09 16:03:32,LilacLight,Slegi HI-W b46-0,"-1997,9375","-624,03125","-2619,25",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","17,7",N
+2022-09-09 16:05:35,h4cky,Oochorrs IJ-C c15-0,"658,1875","-340,8125","-1699,1875",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","25,6",N
+2022-09-09 16:08:24,EREBUS5,Slegi FE-L c23-0,"-1943,34375","-619,96875","-2624,28125",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","60,7",N
+2022-09-09 16:09:22,LilacLight,Slegi FX-X b45-0,"-2000,53125","-643,4375","-2639,125",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","14,8",N
+2022-09-09 16:09:46,Rogixd,Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625",Slegi BT-M c22-0,"-2003,0625","-627,78125","-2640,9375","21,1",Y
+2022-09-09 16:12:08,FULGEN,Slegi JD-I d10-5,"-2032,40625","-622,21875","-2673,71875",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","61,5",N
+2022-09-09 16:12:46,Ralle Randale,Maia,"-81,78125","-149,4375","-343,375",Pleiades Sector EL-X b1-0,"-62,125","-160,625","-403,96875","64,7",N
+2022-09-09 16:14:55,LilacLight,Slegi BT-M c22-1,"-1990,75","-650,375","-2638,875",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","19,8",N
+2022-09-09 16:22:37,Rogixd,Slegi GS-X b45-0,"-2016,65625","-654,6875","-2637,65625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","25,1",N
+2022-09-09 16:23:06,LilacLight,Slegi FX-X b45-0,"-2000,53125","-643,4375","-2639,125",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","14,8",N
+2022-09-09 16:29:56,BL1P,Pleiades Sector HC-T b4-0,"-43,25","-60,03125","-343,03125",Pleiades Sector CQ-Y d58,"-53,5625","-60,9375","-353,03125","14,4",Y
+2022-09-09 16:52:42,Helmut Grokenberger,Slegi HI-W b46-0,"-1997,9375","-624,03125","-2619,25",Slegi FX-X b45-0,"-2000,53125","-643,4375","-2639,125","27,9",N
+2022-09-09 17:27:58,ordınary man,Slegi FX-X b45-0,"-2000,53125","-643,4375","-2639,125",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","14,8",N
+2022-09-09 17:52:35,Gaesatae,Pleiades Sector NI-S b4-2,"-66,4375","-131,375","-336,21875",Merope,"-78,59375","-149,625","-340,53125","22,3",N
+2022-09-09 18:37:48,Stukjes,Slegi GS-X b45-0,"-2016,65625","-654,6875","-2637,65625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","25,1",N
+2022-09-09 18:42:48,Will keaton,Slegi FZ-K c23-0,"-1999,6875","-639,65625","-2596,625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625",28,N
+2022-09-09 18:52:52,Stukjes,Slegi GS-X b45-0,"-2016,65625","-654,6875","-2637,65625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","25,1",N
+2022-09-09 19:06:59,Stukjes,Slegi GS-X b45-0,"-2016,65625","-654,6875","-2637,65625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","25,1",N
+2022-09-09 19:13:32,Stukjes,Slegi GS-X b45-0,"-2016,65625","-654,6875","-2637,65625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","25,1",N
+2022-09-09 19:20:36,Stukjes,Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625",Slegi FX-X b45-0,"-2000,53125","-643,4375","-2639,125","14,8",Y
+2022-09-09 19:22:48,Kai Pelzel,Oochorrs QE-O d7-0,"580,1875","-343,71875","-1695,375",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","54,7",
+2022-09-09 19:24:13,Stukjes,Slegi FX-X b45-0,"-2000,53125","-643,4375","-2639,125",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","14,8",N
+2022-09-09 19:33:21,Will keaton,Slegi GS-X b45-0,"-2016,65625","-654,6875","-2637,65625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","25,1",N
+2022-09-09 20:02:24,Mass1nhibited,HIP 18390,"-299,0625","-229,25","-876,125",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375","22,2",N
+2022-09-09 20:19:34,mxpower76,Oochorrs SZ-N d7-4,"637,5",-349,"-1676,53125",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","24,1",
+2022-09-09 20:22:30,Will keaton,Slegi FX-X b45-0,"-2000,53125","-643,4375","-2639,125",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","14,8",N
+2022-09-09 20:24:15,Denis Page,Slegi HX-X b45-0,"-1953,875","-635,90625","-2632,8125",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","47,5",N
+2022-09-09 20:32:29,Senoreggwardo,Slegi GS-X b45-0,"-2016,65625","-654,6875","-2637,65625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","25,1",N
+2022-09-09 20:43:38,Molan Ryke,Slegi GZ-K c23-1,"-1966,59375","-661,59375","-2616,03125",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","40,6",N
+2022-09-09 20:51:55,Mass1nhibited,HIP 18390,"-299,0625","-229,25","-876,125",California Sector LC-V c2-2,"-312,59375","-226,28125","-878,5","14,1",N
+2022-09-09 20:56:18,Mass1nhibited,California Sector LC-V c2-2,"-312,59375","-226,28125","-878,5",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375","12,8",N
+2022-09-09 21:12:56,h4cky,Slegi HI-W b46-0,"-1997,9375","-624,03125","-2619,25",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","17,7",N
+2022-09-09 21:31:04,Mass1nhibited,HIP 18390,"-299,0625","-229,25","-876,125",California Sector LC-V c2-2,"-312,59375","-226,28125","-878,5","14,1",N
+2022-09-09 21:36:09,Thommychen,Hyades Sector DL-X b1-3,-27,"-90,84375",-235,Pleiades Sector NN-T c3-1,"-34,03125","-107,5625","-270,09375","39,5",N
+2022-09-09 21:46:31,Alfredo Cavatelli,Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625",Slegi FX-X b45-0,"-2000,53125","-643,4375","-2639,125","14,8",Y
+2022-09-09 21:58:10,Alfredo Cavatelli,Slegi GX-X b45-0,"-1981,09375","-642,78125","-2643,5",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","27,1",N
+2022-09-09 22:05:39,Maxwell Hauser [GPL],Slegi HS-X b45-0,"-1998,9375","-655,375","-2638,71875",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","20,4",N
+2022-09-09 22:25:20,Eckee,Slegi GS-X b45-0,"-2016,65625","-654,6875","-2637,65625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","25,1",N
+2022-09-09 23:11:42,SmileyDank,Col 285 Sector HV-W b2-5,"-42,0625","-62,28125","-287,84375",Synuefe LD-A c17-2,"-23,0625","-64,375","-341,34375","56,8",
+2022-09-10 01:09:19,Grimscrub,HIP 17692,"-74,125","-143,96875","-328,78125",Maia,"-81,78125","-149,4375","-343,375","17,4",N
+2022-09-10 01:13:10,Grimscrub,Maia,"-81,78125","-149,4375","-343,375",Celaeno,"-81,09375","-148,3125","-337,09375","6,4",N
+2022-09-10 01:24:53,Historia-g,Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625",Slegi FX-X b45-0,"-2000,53125","-643,4375","-2639,125","14,8",Y
+2022-09-10 01:30:19,EREBUS5,Slegi FX-X b45-0,"-2000,53125","-643,4375","-2639,125",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","14,8",N
+2022-09-10 01:43:18,Sindri Völuspa,Musca Dark Region CQ-Y d86,"423,15625","9,9375","272,65625",Musca Dark Region KI-R b5-5,"435,1875","17,375","273,375","14,2",N
+2022-09-10 02:10:46,Grimscrub,Sterope II,"-81,65625","-147,28125","-340,84375",Pleiades Sector TO-Q b5-1,"-44,28125","-138,09375","-316,3125","45,6",N
+2022-09-10 02:53:22,Louis Calvert,Slegi GS-X b45-0,"-2016,65625","-654,6875","-2637,65625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","25,1",N
+2022-09-10 03:55:01,BL1P,HIP 23022,"-30,375","-51,1875","-338,15625",HIP 22711,"-35,75","-60,84375","-366,90625","30,8",Y
+2022-09-10 04:24:35,RJolley42,Oochorrs SZ-N d7-5,"642,625","-345,5","-1676,125",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,1",N
+2022-09-10 04:30:27,BL1P,HIP 23022,"-30,375","-51,1875","-338,15625",Pleiades Sector DG-X c1-11,"-49,25","-70,4375","-360,8125","35,2",Y
+2022-09-10 04:38:46,BL1P,Pleiades Sector DG-X c1-11,"-49,25","-70,4375","-360,8125",HIP 23022,"-30,375","-51,1875","-338,15625","35,2",Y
+2022-09-10 04:41:50,BL1P,HIP 23022,"-30,375","-51,1875","-338,15625",HIP 22711,"-35,75","-60,84375","-366,90625","30,8",Y
+2022-09-10 04:42:59,DøømHåmstër,Pleiades Sector JH-V c2-2,"-43,03125","-111,34375","-324,875",Pleiades Sector NY-Q b5-1,"-67,5625","-93,96875","-309,5625","33,7",N
+2022-09-10 04:47:14,BL1P,HIP 23022,"-30,375","-51,1875","-338,15625",HIP 22711,"-35,75","-60,84375","-366,90625","30,8",Y
+2022-09-10 05:20:37,BL1P,Synuefe DL-E a66-2,"-46,09375","-41,15625","-364,625",HIP 21852,"-38,53125","-75,3125","-352,40625","37,1",Y
+2022-09-10 05:57:32,LilacLight,Slegi SP-E d12-5,"-1977,1875","-651,375","-2581,5625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625",50,N
+2022-09-10 06:27:14,taytortot,Slegi FE-L c23-1,"-1942,65625","-617,25",-2623,Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","62,4",N
+2022-09-10 07:47:52,Neutronic1,Struve's Lost Sector KH-V c2-8,"38,34375","-183,1875","-429,0625",Pleiades Sector LR-V b2-3,-1,"-155,6875","-379,9375","68,7",N
+2022-09-10 08:00:05,Stukjes,Slegi FX-X b45-0,"-2000,53125","-643,4375","-2639,125",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","14,8",N
+2022-09-10 08:02:19,Stukjes,Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625",Slegi FX-X b45-0,"-2000,53125","-643,4375","-2639,125","14,8",Y
+2022-09-10 08:16:55,Yeri-san,Pleiades Sector IH-V c2-14,"-88,03125","-142,21875","-338,875",Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875","13,2",N
+2022-09-10 08:37:49,Stukjes,Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625",Slegi FX-X b45-0,"-2000,53125","-643,4375","-2639,125","14,8",Y
+2022-09-10 08:47:59,Stukjes,Slegi FX-X b45-0,"-2000,53125","-643,4375","-2639,125",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","14,8",N
+2022-09-10 09:00:18,Stukjes,Slegi FX-X b45-0,"-2000,53125","-643,4375","-2639,125",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","14,8",N
+2022-09-10 09:05:46,Stukjes,Slegi FX-X b45-0,"-2000,53125","-643,4375","-2639,125",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","14,8",N
+2022-09-10 09:15:52,Stukjes,Slegi FX-X b45-0,"-2000,53125","-643,4375","-2639,125",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","14,8",N
+2022-09-10 09:18:31,Stukjes,Slegi GS-X b45-0,"-2016,65625","-654,6875","-2637,65625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","25,1",N
+2022-09-10 09:20:42,Stukjes,Slegi FX-X b45-0,"-2000,53125","-643,4375","-2639,125",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","14,8",N
+2022-09-10 09:23:06,h4cky,Slegi MD-W b46-0,"-1941,4375","-644,375","-2624,6875",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","59,1",N
+2022-09-10 09:40:48,Theory Madness,Slegi EX-X b45-0,"-2014,28125","-637,84375","-2643,125",Slegi HS-X b45-0,"-1998,9375","-655,375","-2638,71875","23,7",N
+2022-09-10 10:06:11,SharkBiscuit,Delphi,"-63,59375","-147,40625","-319,09375",Asterope,"-80,15625","-144,09375","-333,375","22,1",
+2022-09-10 10:15:37,ordınary man,Slegi SP-E d12-5,"-1977,1875","-651,375","-2581,5625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625",50,N
+2022-09-10 10:32:29,ordınary man,Slegi GX-X b45-0,"-1981,09375","-642,78125","-2643,5",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","27,1",N
+2022-09-10 12:55:31,h4cky,Slegi SP-E d12-5,"-1977,1875","-651,375","-2581,5625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625",50,N
+2022-09-10 13:16:11,Cordon Bleu,Oochorrs TZ-N d7-4,"682,6875","-357,25","-1676,34375",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","54,6",N
+2022-09-10 13:21:10,Flip the Great,Oochorrs SZ-N d7-5,"642,625","-345,5","-1676,125",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,1",N
+2022-09-10 14:12:46,DeMortigan,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",Pleiades Sector KC-V c2-9,"-88,71875","-147,8125","-339,0625","2,3",
+2022-09-10 14:38:22,M. V. Coehoorn,Oochorrs SZ-N d7-5,"642,625","-345,5","-1676,125",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","26,1",
+2022-09-10 15:22:01,Dark3982,Musca Dark Region KD-R b5-2,"406,96875","2,625","262,84375",HIP 62270,"413,75","3,46875","264,6875","7,1",N
+2022-09-10 15:27:06,Dark3982,Musca Dark Region HM-V c2-29,"418,46875","-4,5625","270,65625",Musca Dark Region HM-V c2-37,"417,71875","-1,96875","275,375","5,4",N
+2022-09-10 15:49:28,Dark3982,Musca Dark Region HM-V c2-32,"438,09375","4,15625","284,0625",Musca Dark Region CQ-Y d151,"442,375","4,5625","284,4375","4,3",N
+2022-09-10 15:57:16,Dark3982,Musca Dark Region QJ-P b6-5,"453,1875","-3,6875","292,6875",Musca Dark Region HM-V c2-20,"450,125","2,3125","293,3125","6,8",N
+2022-09-10 16:08:20,Dark3982,Musca Dark Region HM-V c2-20,"450,125","2,3125","293,3125",Musca Dark Region KD-R b5-2,"406,96875","2,625","262,84375","52,8",N
+2022-09-10 16:09:05,redundo,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector HR-W d1-36,"-67,65625","-150,71875","-333,3125","14,1",N
+2022-09-10 16:16:11,Dark3982,Musca Dark Region KD-R b5-2,"406,96875","2,625","262,84375",Coalsack Sector KH-V c2-20,"480,125","19,6875","287,8125","79,2",N
+2022-09-10 16:22:51,Dark3982,Musca Dark Region CQ-Y d86,"423,15625","9,9375","272,65625",Musca Dark Region KD-R b5-2,"406,96875","2,625","262,84375","20,3",N
+2022-09-10 16:53:51,DeMortigan,HIP 17862,"-81,4375","-151,90625","-359,59375",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125","21,5",
+2022-09-10 17:25:39,koishukaze,Slegi SP-E d12-5,"-1977,1875","-651,375","-2581,5625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625",50,N
+2022-09-10 17:39:15,SAWAMURA_ERIRI,Slegi GS-X b45-0,"-2016,65625","-654,6875","-2637,65625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","25,1",N
+2022-09-10 18:05:05,Yeri-san,Asterope,"-80,15625","-144,09375","-333,375",Celaeno,"-81,09375","-148,3125","-337,09375","5,7",N
+2022-09-10 18:11:31,Yeri-san,Asterope,"-80,15625","-144,09375","-333,375",Celaeno,"-81,09375","-148,3125","-337,09375","5,7",N
+2022-09-10 18:15:06,Molan Ryke,Slegi SP-E d12-5,"-1977,1875","-651,375","-2581,5625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625",50,N
+2022-09-10 18:42:06,Yeri-san,Celaeno,"-81,09375","-148,3125","-337,09375",Asterope,"-80,15625","-144,09375","-333,375","5,7",N
+2022-09-10 18:43:35,pokegustavo,HR 1554,"-32,375","-57,53125","-340,65625",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","16,9",
+2022-09-10 18:44:39,Oldcarsmell,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",Col 285 Sector AD-Z a2-1,"-42,78125","-40,46875","-320,53125","37,3",
+2022-09-10 18:45:55,pokegustavo,HR 1554,"-32,375","-57,53125","-340,65625",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","16,9",
+2022-09-10 18:51:45,Oldcarsmell,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",Col 285 Sector AD-Z a2-1,"-42,78125","-40,46875","-320,53125","37,3",
+2022-09-10 19:19:10,Yeri-san,Celaeno,"-81,09375","-148,3125","-337,09375",Asterope,"-80,15625","-144,09375","-333,375","5,7",N
+2022-09-10 20:25:47,Sindri Völuspa,Coalsack Sector GW-W d1-75,"425,65625","58,46875","302,78125",Coalsack Sector VU-O b6-6,"418,65625","24,25","304,5625",35,N
+2022-09-10 20:40:47,Sindri Völuspa,Musca Dark Region PJ-P b6-10,"425,09375","-0,28125","286,9375",Musca Dark Region GO-E a13-0,"424,15625","-3,625","286,78125","3,5",N
+2022-09-10 21:22:01,pokegustavo,Slegi FX-X b45-0,"-2000,53125","-643,4375","-2639,125",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625","14,8",
+2022-09-10 21:25:50,SAWAMURA_ERIRI,Pleiades Sector AL-X b1-0,"-141,40625","-149,5","-391,78125",Sterope II,"-81,65625","-147,28125","-340,84375","78,5",N
+2022-09-10 22:59:22,DøømHåmstër,Pleiades Sector FB-X c1-3,"-43,4375","-140,96875","-373,96875",Pleiades Sector GW-W c1-13,"-77,375","-156,3125","-352,03125","43,2",N
+2022-09-10 23:05:01,HaLfY47,Slegi SP-E d12-5,"-1977,1875","-651,375","-2581,5625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625",50,N
+2022-09-10 23:24:25,Hisaki51,Synuefe WG-A b34-3,"-9,875","-56,3125","-334,34375",HIP 22460,"-41,3125","-58,96875","-354,78125","37,6",Y
+2022-09-11 00:07:08,patmarsons,Slegi SP-E d12-5,"-1977,1875","-651,375","-2581,5625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625",50,N
+2022-09-11 00:59:24,redundo,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector HR-W d1-36,"-67,65625","-150,71875","-333,3125","14,1",N
+2022-09-11 01:27:02,Arsinoe,Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",16,N
+2022-09-11 01:46:01,Sayaka,California Sector HW-W c1-6,"-325,59375","-236,09375","-929,1875",California Sector TT-R b4-0,"-331,09375","-247,0625","-913,125","20,2",N
+2022-09-11 02:48:50,Mach10z,Pleiades Sector HR-W d1-79,"-80,625","-146,65625","-343,25",Pleiades Sector IH-V c2-0,"-104,3125","-140,4375","-344,78125","24,5",N
+2022-09-11 03:52:59,Rampant Fox,Musca Dark Region QJ-P b6-3,"447,0625","-3,5","277,46875",Musca Dark Region PJ-P b6-1,"432,625","2,53125","288,6875","19,3",N
+2022-09-11 04:18:24,_yellowcavefrog,HIP 17692,"-74,125","-143,96875","-328,78125",Asterope,"-80,15625","-144,09375","-333,375","7,6",N
+2022-09-11 04:25:37,Rampant Fox,Musca Dark Region PJ-P b6-1,"432,625","2,53125","288,6875",Musca Dark Region BQ-Y d123,"376,90625","-0,84375","259,96875","62,8",N
+2022-09-11 04:31:48,Louis Calvert,Pleiades Sector DW-V b2-1,"-124,59375","-133,15625","-383,21875",Pleiades Sector HC-U b3-0,"-107,46875","-140,53125","-362,375",28,N
+2022-09-11 04:34:48,Louis Calvert,Pleiades Sector HC-U b3-0,"-107,46875","-140,53125","-362,375",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","37,1",N
+2022-09-11 05:32:30,BL1P,Slegi FZ-K c23-0,"-1999,6875","-639,65625","-2596,625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625",28,N
+2022-09-11 06:34:13,tokisu,Pleiades Sector LY-Q b5-2,"-121,59375","-85,78125","-306,34375",Pleiades Sector MI-S b4-1,"-103,375","-138,3125","-339,8125","64,9",N
+2022-09-11 08:21:00,Marvin Jones,Synuefe VG-A b34-3,"-35,71875","-51,65625","-330,15625",HIP 22460,"-41,3125","-58,96875","-354,78125","26,3",Y
+2022-09-11 08:24:37,Marvin Jones,Synuefe VG-A b34-3,"-35,71875","-51,65625","-330,15625",HIP 22460,"-41,3125","-58,96875","-354,78125","26,3",Y
+2022-09-11 08:27:16,Galactik,Slegi SP-E d12-5,"-1977,1875","-651,375","-2581,5625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625",50,N
+2022-09-11 09:17:05,BL1P,Slegi FZ-K c23-0,"-1999,6875","-639,65625","-2596,625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625",28,N
+2022-09-11 09:28:37,Will keaton,Arietis Sector UK-M a8-2,"-69,0625","-42,65625","-132,78125",HIP 15329,"-87,84375",-52,"-167,375","40,5",N
+2022-09-11 09:32:24,Anarril,Pleiades Sector XP-W b2-0,"-84,625","-62,34375","-384,53125",Synuefe CT-O d7-57,"-64,09375","-45,40625","-349,90625","43,7",
+2022-09-11 09:52:56,Neutronic1,Synuefe RA-C b33-0,"-25,875","-50,5","-364,125",HIP 22460,"-41,3125","-58,96875","-354,78125","19,9",Y
+2022-09-11 10:19:17,Neutronic1,HIP 22410,"-49,46875","-55,25","-360,59375",HIP 22460,"-41,3125","-58,96875","-354,78125","10,7",Y
+2022-09-11 10:44:26,Anarril,Pleiades Sector XP-W b2-0,"-84,625","-62,34375","-384,53125",Synuefe CT-O d7-57,"-64,09375","-45,40625","-349,90625","43,7",
+2022-09-11 10:44:40,Anarril,Pleiades Sector XP-W b2-0,"-84,625","-62,34375","-384,53125",Synuefe CT-O d7-57,"-64,09375","-45,40625","-349,90625","43,7",
+2022-09-11 10:50:52,Anarril,Pleiades Sector XP-W b2-0,"-84,625","-62,34375","-384,53125",Synuefe CT-O d7-57,"-64,09375","-45,40625","-349,90625","43,7",
+2022-09-11 10:51:24,Anarril,Pleiades Sector XP-W b2-0,"-84,625","-62,34375","-384,53125",Synuefe CT-O d7-57,"-64,09375","-45,40625","-349,90625","43,7",
+2022-09-11 10:51:42,Anarril,Pleiades Sector XP-W b2-0,"-84,625","-62,34375","-384,53125",Synuefe CT-O d7-57,"-64,09375","-45,40625","-349,90625","43,7",
+2022-09-11 10:52:06,Anarril,Pleiades Sector XP-W b2-0,"-84,625","-62,34375","-384,53125",Synuefe CT-O d7-57,"-64,09375","-45,40625","-349,90625","43,7",
+2022-09-11 10:52:29,Anarril,Pleiades Sector XP-W b2-0,"-84,625","-62,34375","-384,53125",Synuefe CT-O d7-57,"-64,09375","-45,40625","-349,90625","43,7",
+2022-09-11 12:01:49,ProCactus167,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","4,4",N
+2022-09-11 12:29:43,LilacLight,Oochorrs SZ-N d7-4,"637,5",-349,"-1676,53125",Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625","24,1",N
+2022-09-11 12:33:16,ProCactus167,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Asterope,"-80,15625","-144,09375","-333,375","4,4",N
+2022-09-11 12:44:19,LilacLight,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625",Oochorrs SZ-N d7-4,"637,5",-349,"-1676,53125","24,1",Y
+2022-09-11 13:01:26,LilacLight,Oochorrs SZ-N d7-4,"637,5",-349,"-1676,53125",Oochorrs SZ-N d7-5,"642,625","-345,5","-1676,125","6,2",N
+2022-09-11 13:01:43,Louis Calvert,Asterope,"-80,15625","-144,09375","-333,375",Merope,"-78,59375","-149,625","-340,53125","9,2",N
+2022-09-11 13:20:20,LilacLight,Oochorrs LP-A c16-0,"616,1875","-341,9375","-1664,90625",Oochorrs SZ-N d7-4,"637,5",-349,"-1676,53125","25,3",N
+2022-09-11 14:13:48,Cate Nicholls,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625",Oochorrs SZ-N d7-5,"642,625","-345,5","-1676,125","26,1",Y
+2022-09-11 14:23:49,Reyedog,Slegi FZ-K c23-0,"-1999,6875","-639,65625","-2596,625",Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625",28,N
+2022-09-11 15:39:13,Roz Daemon,Maia,"-81,78125","-149,4375","-343,375",Pleiades Sector YA-N b7-3,"-85,84375","-139,3125","-281,71875","62,6",
+2022-09-11 17:03:26,tokisu,Pleiades Sector PN-T b3-0,"-79,53125","-199,9375","-361,59375",Pleiades Sector WE-Q b5-1,"-46,5625","-183,65625","-308,625","64,5",N
+2022-09-11 17:06:46,LilacLight,Slegi SP-E d12-6,"-1974,46875","-651,15625","-2577,4375",Slegi SP-E d12-5,"-1977,1875","-651,375","-2581,5625","4,9",N
+2022-09-11 17:13:11,LilacLight,Slegi SP-E d12-6,"-1974,46875","-651,15625","-2577,4375",Slegi SP-E d12-0,"-1974,96875","-656,65625","-2578,5625","5,6",N
+2022-09-11 17:15:15,Historia-g,Slegi JD-W b46-0,"-2000,40625","-640,75","-2624,5625",Slegi SP-E d12-6,"-1974,46875","-651,15625","-2577,4375","54,8",Y
+2022-09-11 17:16:35,Ecthelion,Merope,"-78,59375","-149,625","-340,53125",Maia,"-81,78125","-149,4375","-343,375","4,3",N
+2022-09-11 17:19:58,BL1P,Slegi SP-E d12-5,"-1977,1875","-651,375","-2581,5625",Slegi SP-E d12-6,"-1974,46875","-651,15625","-2577,4375","4,9",Y
+2022-09-11 17:23:10,BL1P,Slegi SP-E d12-6,"-1974,46875","-651,15625","-2577,4375",Slegi SP-E d12-0,"-1974,96875","-656,65625","-2578,5625","5,6",N
+2022-09-11 17:26:25,LilacLight,Slegi KF-J c24-1,"-1957,84375","-655,6875","-2565,375",Slegi SP-E d12-0,"-1974,96875","-656,65625","-2578,5625","21,6",N
+2022-09-11 17:46:03,quinnrz,Slegi SP-E d12-5,"-1977,1875","-651,375","-2581,5625",Slegi SP-E d12-6,"-1974,46875","-651,15625","-2577,4375","4,9",N
+2022-09-11 17:48:56,BL1P,Slegi SP-E d12-0,"-1974,96875","-656,65625","-2578,5625",Slegi SP-E d12-5,"-1977,1875","-651,375","-2581,5625","6,5",N
+2022-09-11 17:52:38,BL1P,Slegi SP-E d12-0,"-1974,96875","-656,65625","-2578,5625",Slegi SP-E d12-5,"-1977,1875","-651,375","-2581,5625","6,5",N
+2022-09-11 17:56:14,LilacLight,Slegi KF-J c24-1,"-1957,84375","-655,6875","-2565,375",Slegi SP-E d12-6,"-1974,46875","-651,15625","-2577,4375",21,N
+2022-09-11 17:56:55,quinnrz,Slegi SP-E d12-6,"-1974,46875","-651,15625","-2577,4375",Slegi SP-E d12-5,"-1977,1875","-651,375","-2581,5625","4,9",N
+2022-09-11 18:06:21,BL1P,Slegi SP-E d12-6,"-1974,46875","-651,15625","-2577,4375",Slegi SP-E d12-5,"-1977,1875","-651,375","-2581,5625","4,9",N
+2022-09-11 18:21:34,FULGEN,Slegi SP-E d12-5,"-1977,1875","-651,375","-2581,5625",Slegi SP-E d12-6,"-1974,46875","-651,15625","-2577,4375","4,9",Y
+2022-09-11 18:27:23,quinnrz,Slegi SP-E d12-6,"-1974,46875","-651,15625","-2577,4375",Slegi SP-E d12-5,"-1977,1875","-651,375","-2581,5625","4,9",N
+2022-09-11 18:43:45,quinnrz,Slegi SP-E d12-5,"-1977,1875","-651,375","-2581,5625",Slegi SP-E d12-6,"-1974,46875","-651,15625","-2577,4375","4,9",N
+2022-09-11 19:07:11,Slipi,Slegi CC-P b50-0,-1945,"-643,40625","-2540,53125",Slegi GI-N b51-0,"-1938,28125","-628,5","-2523,5","23,6",N
+2022-09-11 19:12:24,LilacLight,Slegi NQ-H c25-1,"-1939,90625",-610,"-2521,59375",Slegi GI-N b51-0,"-1938,28125","-628,5","-2523,5","18,7",N
+2022-09-11 19:32:15,_yellowcavefrog,Pleiades Sector RO-Q b5-3,"-70,46875","-131,8125","-306,78125",Pleiades Sector XF-N b7-3,"-69,3125","-112,9375","-277,59375","34,8",N
+2022-09-11 19:36:10,_yellowcavefrog,Pleiades Sector RO-Q b5-2,"-67,03125","-136,5625","-308,09375",Pleiades Sector NN-T c3-13,"-62,5625","-129,9375","-271,4375","37,5",N
+2022-09-11 19:46:23,Mallchad,Slegi KF-J c24-1,"-1957,84375","-655,6875","-2565,375",Slegi GI-N b51-0,"-1938,28125","-628,5","-2523,5","53,6",N
+2022-09-11 20:04:30,Slipi,Slegi OL-H c25-0,"-1972,09375","-640,6875","-2534,75",Slegi GI-N b51-0,"-1938,28125","-628,5","-2523,5","37,7",N
+2022-09-11 20:30:02,Rogixd,Slegi CC-P b50-0,-1945,"-643,40625","-2540,53125",Slegi GI-N b51-0,"-1938,28125","-628,5","-2523,5","23,6",N
+2022-09-11 21:15:51,LilacLight,Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","15,2",N
+2022-09-11 21:17:03,Gray Z7Z,Pleiades Sector KC-V c2-4,"-88,8125","-148,71875","-321,1875",Pleiades Sector IH-V c2-7,"-78,46875","-144,9375","-324,9375","11,6",N
+2022-09-11 21:27:33,LilacLight,Slegi JY-L b52-0,"-1880,46875","-602,09375","-2503,21875",Slegi LT-L b52-0,"-1881,09375","-621,90625","-2502,78125","19,8",N
+2022-09-11 21:32:37,Wotherspoon,Oochorrs SZ-N d7-1,"575,5625","-373,375","-1699,78125",Oochorrs SZ-N d7-4,"637,5",-349,"-1676,53125","70,5",N
+2022-09-11 21:52:37,LilacLight,Slegi IY-L b52-0,"-1898,15625","-597,875","-2503,59375",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","21,9",N
+2022-09-11 22:31:14,quinnrz,Slegi PZ-J b53-0,"-1884,3125","-607,1875",-2475,Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","17,6",N
+2022-09-11 22:38:03,niko redman,Oochorrs JE-C c15-0,"634,25","-349,9375","-1700,40625",Oochorrs SZ-N d7-5,"642,625","-345,5","-1676,125","26,1",
+2022-09-11 22:47:57,quinnrz,Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","15,2",N
+2022-09-11 23:28:07,quinnrz,Slegi LT-L b52-0,"-1881,09375","-621,90625","-2502,78125",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375",21,N
+2022-09-11 23:34:25,quinnrz,Slegi IY-L b52-0,"-1898,15625","-597,875","-2503,59375",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","21,9",N
+2022-09-11 23:39:28,Will keaton,Slegi LT-L b52-0,"-1881,09375","-621,90625","-2502,78125",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375",21,N
+2022-09-11 23:41:27,Firehawk894,Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","15,2",
+2022-09-11 23:41:34,quinnrz,Slegi JY-L b52-0,"-1880,46875","-602,09375","-2503,21875",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","22,7",N
+2022-09-11 23:50:15,Will keaton,Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625",Slegi IY-L b52-0,"-1898,15625","-597,875","-2503,59375","34,6",N
+2022-09-11 23:55:28,Firehawk894,Slegi WC-E c27-1,"-1900,875","-623,375","-2462,34375",Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625","18,3",
+2022-09-12 00:08:25,quinnrz,Slegi NE-K b53-0,"-1865,5625","-600,875","-2484,5",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","31,1",N
+2022-09-12 00:26:00,Chewcat1,Slegi OZ-E d12-9,"-1968,03125","-444,03125","-2564,375",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","199,7",N
+2022-09-12 00:28:09,Will keaton,Slegi HI-N b51-0,"-1920,6875","-639,75","-2519,0625",Slegi PZ-J b53-0,"-1884,3125","-607,1875",-2475,"65,8",N
+2022-09-12 00:34:56,quinnrz,Slegi WC-E c27-1,"-1900,875","-623,375","-2462,34375",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375",29,N
+2022-09-12 00:55:22,quinnrz,Slegi WC-E c27-0,"-1904,03125","-621,40625","-2461,875",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","29,8",N
+2022-09-12 01:02:55,REBECA ASIMOV,Suluo,"381,125","-384,375","-725,84375",Witch Head Sector IH-V c2-7,"363,5625","-380,78125","-699,375",32,N
+2022-09-12 01:03:30,Kurataki,Oochorrs RE-O d7-4,"668,96875","-311,9375","-1640,875",Oochorrs SZ-N d7-5,"642,625","-345,5","-1676,125","55,3",N
+2022-09-12 01:20:41,Will keaton,Slegi LJ-K b53-0,"-1880,03125","-581,34375","-2480,03125",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","35,7",N
+2022-09-12 01:31:06,Mallchad,Slegi GI-N b51-0,"-1938,28125","-628,5","-2523,5",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","58,6",N
+2022-09-12 02:12:11,REBECA ASIMOV,Pleiades Sector NX-T b3-2,"-43,6875","-162,28125","-361,28125",Pleiades Sector GW-W c1-15,"-76,9375","-153,71875","-346,625","37,3",N
+2022-09-12 02:25:55,REBECA ASIMOV,Pleiades Sector GW-W c1-15,"-76,9375","-153,71875","-346,625",Maia,"-81,78125","-149,4375","-343,375","7,2",N
+2022-09-12 03:02:54,quinnrz,Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","15,2",N
+2022-09-12 03:30:51,quinnrz,Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","15,2",N
+2022-09-12 03:36:17,quinnrz,Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","15,2",N
+2022-09-12 03:42:51,quinnrz,Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","15,2",N
+2022-09-12 03:55:53,quinnrz,Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375",Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625","15,2",Y
+2022-09-12 04:20:41,quinnrz,Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375",Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625","15,2",Y
+2022-09-12 04:30:01,quinnrz,Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","15,2",N
+2022-09-12 04:55:54,quinnrz,Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375",Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625","15,2",Y
+2022-09-12 05:07:12,quinnrz,Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","15,2",N
+2022-09-12 06:46:15,Sayaka,California Sector HW-W c1-4,"-330,71875","-233,59375","-909,1875",California Sector UJ-Q b5-0,"-319,6875","-222,3125","-899,9375","18,3",N
+2022-09-12 07:29:50,CJMVAUCLUSE,Pleiades Sector HC-T b4-0,"-43,25","-60,03125","-343,03125",HIP 22460,"-41,3125","-58,96875","-354,78125",12,Y
+2022-09-12 10:00:10,nixon12821,Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375",Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625","15,2",Y
+2022-09-12 10:12:37,nixon12821,Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","15,2",N
+2022-09-12 10:23:31,nixon12821,Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","15,2",N
+2022-09-12 10:43:23,ProCactus167,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Asterope,"-80,15625","-144,09375","-333,375","4,4",N
+2022-09-12 10:59:40,LilacLight,Slegi JY-L b52-0,"-1880,46875","-602,09375","-2503,21875",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","22,7",N
+2022-09-12 13:01:41,Sayaka,California Sector KH-V c2-4,"-290,125","-211,875","-872,8125",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","50,6",N
+2022-09-12 13:50:52,LilacLight,Oochost WU-S c6-0,"-436,4375","-186,6875","-2038,1875",Oochost XE-X d2-6,"-425,34375","-186,78125","-2043,625","12,4",Y
+2022-09-12 14:15:38,dko,Oochorrs PV-Y c16-0,"620,75","-341,75","-1622,40625",Oochorrs SZ-N d7-5,"642,625","-345,5","-1676,125","58,1",N
+2022-09-12 14:18:56,LilacLight,Oochost XE-X d2-6,"-425,34375","-186,78125","-2043,625",Oochost WU-S c6-0,"-436,4375","-186,6875","-2038,1875","12,4",N
+2022-09-12 14:37:23,Gray Z7Z,Pleiades Sector PD-S b4-2,"-75,5625","-152,40625","-336,40625",Sterope II,"-81,65625","-147,28125","-340,84375","9,1",N
+2022-09-12 14:42:27,Castro27,Oochost XE-X d2-6,"-425,34375","-186,78125","-2043,625",Oochost WU-S c6-0,"-436,4375","-186,6875","-2038,1875","12,4",N
+2022-09-12 15:03:47,Grimscrub,HIP 17692,"-74,125","-143,96875","-328,78125",HR 1185,"-64,65625","-148,9375","-330,40625","10,8",N
+2022-09-12 15:10:51,Firedrax,Hyades Sector AQ-X b1-2,"-62,5","-81,28125","-243,46875",Pleiades Sector WK-N b7-0,"-64,25","-102,5625","-270,59375","34,5",N
+2022-09-12 15:52:24,Firedrax,Col 285 Sector PC-T b4-3,"-81,4375",-84,-265,Pleiades Sector VK-N b7-3,"-75,40625","-104,59375",-280,"26,2",N
+2022-09-12 16:14:08,Senoreggwardo,Oochost VJ-X d2-0,"-460,28125","-177,75","-2100,46875",Oochost WU-S c6-0,"-436,4375","-186,6875","-2038,1875","67,3",N
+2022-09-12 16:25:04,quinnrz,Oochost XE-X d2-6,"-425,34375","-186,78125","-2043,625",Oochost WU-S c6-0,"-436,4375","-186,6875","-2038,1875","12,4",N
+2022-09-12 16:35:01,quinnrz,Oochost XE-X d2-6,"-425,34375","-186,78125","-2043,625",Oochost WU-S c6-0,"-436,4375","-186,6875","-2038,1875","12,4",N
+2022-09-12 16:39:29,quinnrz,Oochost XE-X d2-6,"-425,34375","-186,78125","-2043,625",Oochost WU-S c6-0,"-436,4375","-186,6875","-2038,1875","12,4",N
+2022-09-12 16:58:29,quinnrz,Oochost XE-X d2-6,"-425,34375","-186,78125","-2043,625",Oochost WU-S c6-0,"-436,4375","-186,6875","-2038,1875","12,4",N
+2022-09-12 17:02:59,Mariocki,Oochorrs LP-A c16-0,"616,1875","-341,9375","-1664,90625",Oochorrs PV-Y c16-0,"620,75","-341,75","-1622,40625","42,7",N
+2022-09-12 17:30:40,Mariocki,Oochorrs IC-U e3-1,"602,90625","-344,5","-1656,46875",Oochorrs PV-Y c16-0,"620,75","-341,75","-1622,40625","38,6",N
+2022-09-12 18:31:32,Reyedog,Pleiades Sector LD-R b5-4,"-73,46875","-75,625","-315,0625",Pleiades Sector FW-W d1-43,"-77,21875","-70,9375","-283,96875","31,7",N
+2022-09-12 18:40:32,Loustique,Oochorrs QQ-Y c16-1,"587,34375","-372,21875","-1603,28125",Oochorrs PV-Y c16-0,"620,75","-341,75","-1622,40625","49,1",N
+2022-09-12 18:52:30,Firedrax,Pleiades Sector HH-V c2-1,"-139,78125","-137,125","-344,25",HR 1183,"-72,875","-145,09375","-336,96875","67,8",N
+2022-09-12 18:54:06,Loustique,Oochorrs OV-Y c16-0,"599,40625","-323,25","-1610,0625",Oochorrs PV-Y c16-0,"620,75","-341,75","-1622,40625","30,8",N
+2022-09-12 20:01:37,Surcouf87,Mel 22 Sector IN-S c4-2,"-190,40625","-86,6875","-416,4375",Pleiades Sector BG-X c1-13,"-142,28125","-85,71875","-364,1875",71,N
+2022-09-12 20:33:20,IvIePhisto,Oochorrs IC-U e3-1,"602,90625","-344,5","-1656,46875",Oochorrs PV-Y c16-0,"620,75","-341,75","-1622,40625","38,6",N
+2022-09-12 21:04:49,AHappyClownFish,Pleiades Sector IH-V c2-5,"-78,71875","-138,03125","-321,78125",Pleiades Sector HR-W d1-57,"-86,40625","-125,15625","-301,84375","24,9",N
+2022-09-12 21:57:54,Suremaker,Pleiades Sector VO-Z b3,"-83,96875","-123,78125","-411,3125",Pleiades Sector DL-Y d37,"-80,46875","-131,96875","-386,90625",26,N
+2022-09-12 22:01:37,Suremaker,Pleiades Sector DL-Y d37,"-80,46875","-131,96875","-386,90625",Pleiades Sector GW-W c1-7,"-78,25","-149,90625","-376,96875","20,6",N
+2022-09-12 22:04:03,Suremaker,Pleiades Sector GW-W c1-7,"-78,25","-149,90625","-376,96875",Electra,"-86,0625","-159,9375","-361,65625","19,9",N
+2022-09-12 22:30:52,M. V. Coehoorn,Delphi,"-63,59375","-147,40625","-319,09375",Pleiades Sector GW-W c1-5,"-84,65625",-159,"-380,3125","65,8",
+2022-09-12 22:47:44,Wryson Rose,Synuefe LD-A c17-0,"-22,84375","-64,0625","-339,09375",HIP 22460,"-41,3125","-58,96875","-354,78125","24,8",Y
+2022-09-13 00:01:28,Wryson Rose,Pleiades Sector HM-V c2-12,"-38,71875","-104,1875","-332,4375",Synuefe LD-A c17-0,"-22,84375","-64,0625","-339,09375","43,7",N
+2022-09-13 00:40:39,Meekohi,Witch Head Sector BV-Y c0,"389,9375","-354,9375","-750,875",Onoros,"389,71875","-379,9375","-724,15625","36,6",
+2022-09-13 00:54:30,Meekohi,Wellington,"386,15625","-390,75","-692,53125",Witch Head Sector DL-Y d18,"360,875","-407,75","-669,03125","38,5",
+2022-09-13 01:17:43,EREBUS5,Slegi IY-L b52-0,"-1898,15625","-597,875","-2503,59375",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","21,9",N
+2022-09-13 02:13:23,pokegustavo,Pleiades Sector BL-X c1-0,"-60,84375","-64,84375","-384,5625",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","36,9",
+2022-09-13 02:17:23,Trident411,Taurus Dark Region KN-S b4-3,"-71,25","-54,46875","-432,96875",Pleiades Sector CW-U b3-1,"-46,53125","-62,9375","-364,5625","73,2",N
+2022-09-13 04:16:03,LilacLight,Oochost FC-O c9-2,"-342,34375","-89,71875","-1916,6875",Oochost DM-P c8-0,"-421,375","-183,59375","-1982,75","139,4",N
+2022-09-13 06:42:56,CP_Luiz,Pleiades Sector AL-X c1-9,"-82,15625","-54,46875","-359,21875",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","38,8",Y
+2022-09-13 06:55:29,tnks4listening,Celaeno,"-81,09375","-148,3125","-337,09375",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","4,6",N
+2022-09-13 07:10:36,CP_Luiz,Pleiades Sector BG-X c1-0,"-123,6875","-88,46875",-360,Pleiades Sector AL-X c1-6,"-69,21875","-61,625",-356,"60,9",N
+2022-09-13 07:14:23,CP_Luiz,Pleiades Sector AL-X c1-6,"-69,21875","-61,625",-356,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","24,9",Y
+2022-09-13 08:14:11,Will keaton,California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","45,3",N
+2022-09-13 09:11:14,Will keaton,Pleiades Sector KH-V b2-1,"-90,71875","-201,5","-374,96875",Pleiades Sector PN-T b3-0,"-79,53125","-199,9375","-361,59375","17,5",N
+2022-09-13 10:47:30,HaLfY47,Slegi VH-E c27-1,"-1860,25","-582,8125","-2462,15625",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","52,4",N
+2022-09-13 11:12:35,LilacLight,Oochost GH-P c8-0,"-382,5","-193,875","-1975,125",Oochost DM-P c8-0,"-421,375","-183,59375","-1982,75","40,9",N
+2022-09-13 12:25:07,Buur,Maia,"-81,78125","-149,4375","-343,375",Pleiades Sector DG-X c1-15,"-55,09375","-101,65625","-353,375","55,6",N
+2022-09-13 12:27:28,Buur,Pleiades Sector DG-X c1-15,"-55,09375","-101,65625","-353,375",HIP 22460,"-41,3125","-58,96875","-354,78125","44,9",Y
+2022-09-13 12:32:34,IvIePhisto,Oochorrs OV-Y c16-0,"599,40625","-323,25","-1610,0625",Oochorrs PV-Y c16-0,"620,75","-341,75","-1622,40625","30,8",N
+2022-09-13 12:41:29,Grimscrub,Slegi VA-D d13-1,"-1893,625","-575,9375","-2456,15625",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","49,4",N
+2022-09-13 13:56:07,Loustique,Oochorrs OV-Y c16-0,"599,40625","-323,25","-1610,0625",Oochorrs PV-Y c16-0,"620,75","-341,75","-1622,40625","30,8",N
+2022-09-13 15:26:47,Space Mickk,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector QO-Q b5-2,"-90,5625","-136,46875","-316,59375","21,2",N
+2022-09-13 15:59:58,ProCactus167,Pleiades Sector MN-T c3-12,"-70,65625","-119,75","-302,8125",Pleiades Sector IH-V c2-5,"-78,71875","-138,03125","-321,78125","27,6",N
+2022-09-13 16:13:21,ProCactus167,Pleiades Sector IH-V c2-5,"-78,71875","-138,03125","-321,78125",Asterope,"-80,15625","-144,09375","-333,375","13,2",N
+2022-09-13 16:55:37,LilacLight,Oochost DW-T d4-7,"-410,9375","-184,3125","-1919,03125",Oochost DM-P c8-0,"-421,375","-183,59375","-1982,75","64,6",N
+2022-09-13 18:06:45,Loustique,Oochorrs OV-Y c16-0,"599,40625","-323,25","-1610,0625",Oochorrs PV-Y c16-0,"620,75","-341,75","-1622,40625","30,8",N
+2022-09-13 18:43:33,Ecthelion,HIP 17692,"-74,125","-143,96875","-328,78125",Merope,"-78,59375","-149,625","-340,53125","13,8",N
+2022-09-13 18:46:14,LilacLight,Oochost DW-T d4-7,"-410,9375","-184,3125","-1919,03125",Oochost DM-P c8-0,"-421,375","-183,59375","-1982,75","64,6",N
+2022-09-13 19:44:51,LilacLight,Oochost FR-T d4-2,"-425,0625","-203,75","-1925,0625",Oochost DW-T d4-7,"-410,9375","-184,3125","-1919,03125","24,8",N
+2022-09-13 19:53:22,pokegustavo,Col 285 Sector GL-X c1-8,"-25,875","-75,71875",-290,Pleiades Sector GW-W d1-47,"-46,71875","-69,96875","-334,3125","49,3",
+2022-09-13 21:38:49,glaCestus,Oochorrs OV-Y c16-0,"599,40625","-323,25","-1610,0625",Oochorrs PV-Y c16-0,"620,75","-341,75","-1622,40625","30,8",N
+2022-09-13 21:44:44,Amber lovelace,California Sector LX-T b3-0,"-337,90625","-211,9375","-927,03125",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","23,1",N
+2022-09-13 22:17:40,LilacLight,Oochorrs NA-Z c16-0,"620,75","-300,15625","-1624,84375",Oochorrs OV-Y c16-0,"599,40625","-323,25","-1610,0625","34,7",N
+2022-09-13 22:46:15,Alfredo Cavatelli,HIP 17403,"-93,6875","-158,96875","-367,625",HIP 17704,"-72,03125","-133,90625","-311,09375","65,5",N
+2022-09-14 00:03:09,DoG Duggy,Oochost LY-L c10-0,"-421,71875","-181,25","-1899,4375",Oochost DW-T d4-7,"-410,9375","-184,3125","-1919,03125","22,6",N
+2022-09-14 00:07:38,Chewcat1,Oochost DM-P c8-0,"-421,375","-183,59375","-1982,75",Oochost DW-T d4-7,"-410,9375","-184,3125","-1919,03125","64,6",N
+2022-09-14 00:14:32,Chewcat1,Oochost LY-L c10-0,"-421,71875","-181,25","-1899,4375",Oochost DW-T d4-7,"-410,9375","-184,3125","-1919,03125","22,6",N
+2022-09-14 00:46:55,Alfredo Cavatelli,California Sector JH-V c2-11,"-312,875","-217,3125","-891,09375",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","23,4",N
+2022-09-14 02:28:57,Djbeef,Delphi,"-63,59375","-147,40625","-319,09375",Maia,"-81,78125","-149,4375","-343,375","30,4",
+2022-09-14 03:21:34,CP_Luiz,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",Y
+2022-09-14 04:25:29,SurpriseSuprise,Celaeno,"-81,09375","-148,3125","-337,09375",Pleiades Sector TU-O b6-0,"-120,125","-142,03125",-304,"51,6",N
+2022-09-14 07:31:24,Tentacle-Sama,Col 285 Sector DP-Y b1-3,"-36,21875","-59,6875","-311,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","43,6",Y
+2022-09-14 10:51:49,Dan Steele,Pleiades Sector HR-W d1-79,"-80,625","-146,65625","-343,25",Pleiades Sector IH-V c2-5,"-78,71875","-138,03125","-321,78125","23,2",N
+2022-09-14 10:56:18,davider,Maia,"-81,78125","-149,4375","-343,375",Taygeta,"-88,5","-159,6875","-366,28125",26,
+2022-09-14 12:19:45,davider,Pleiades Sector IH-U b3-3,"-50,3125","-108,40625","-347,25",HIP 21852,"-38,53125","-75,3125","-352,40625","35,5",N
+2022-09-14 13:00:02,davider,Col 285 Sector NW-U b3-1,"-44,53125","-79,46875","-281,78125",Pleiades Sector JX-S b4-2,"-41,03125","-80,78125","-340,8125","59,1",N
+2022-09-14 13:59:14,KENSHIRO KASUMI,Hyades Sector ZP-X b1-2,"-81,0625","-76,15625","-241,4375",Pleiades Sector XF-N b7-2,"-81,6875","-124,03125","-276,71875","59,5",N
+2022-09-14 14:41:19,Eagle131,Oochorrs PV-Y c16-0,"620,75","-341,75","-1622,40625",Oochorrs OV-Y c16-0,"599,40625","-323,25","-1610,0625","30,8",N
+2022-09-14 15:49:47,LilacLight,Oochorrs TK-M d8-0,"571,28125","-317,40625","-1599,65625",Oochorrs OV-Y c16-0,"599,40625","-323,25","-1610,0625","30,6",N
+2022-09-14 16:08:07,Super_hulk,Oochost EW-T d4-0,"-382,9375","-176,59375","-1937,15625",Oochost DW-T d4-7,"-410,9375","-184,3125","-1919,03125","34,2",N
+2022-09-14 17:06:09,Stukjes,Slegi IY-L b52-0,"-1898,15625","-597,875","-2503,59375",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","21,9",N
+2022-09-14 17:08:46,Stukjes,Slegi NE-K b53-0,"-1865,5625","-600,875","-2484,5",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","31,1",N
+2022-09-14 17:21:59,Stukjes,Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","15,2",N
+2022-09-14 17:35:17,Stukjes,Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","15,2",N
+2022-09-14 17:38:31,Stukjes,Slegi IY-L b52-0,"-1898,15625","-597,875","-2503,59375",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","21,9",N
+2022-09-14 17:42:58,Stukjes,Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","15,2",N
+2022-09-14 17:49:56,Stukjes,Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","15,2",N
+2022-09-14 18:27:35,Stukjes,Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375",Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625","15,2",Y
+2022-09-14 18:32:07,Stukjes,Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","15,2",N
+2022-09-14 18:39:11,Stukjes,Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","15,2",N
+2022-09-14 18:48:23,Stukjes,Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375",Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625","15,2",Y
+2022-09-14 18:50:47,Stukjes,Slegi OZ-J b53-0,"-1901,6875","-623,5","-2480,625",Slegi KT-L b52-0,"-1893,8125","-613,1875","-2488,59375","15,2",N
+2022-09-14 20:39:20,KENSHIRO KASUMI,Pleione,-77,"-146,78125","-344,125",Pleiades Sector HR-W d1-79,"-80,625","-146,65625","-343,25","3,7",N
+2022-09-14 20:52:47,Alfredo Cavatelli,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector DL-Y d6,"-357,0625","-257,46875","-943,90625",63,N
+2022-09-14 21:35:19,glaCestus,Col 69 Sector GV-X c1-0,"569,0625","-326,5625","-1579,03125",Oochorrs OV-Y c16-0,"599,40625","-323,25","-1610,0625","43,5",N
+2022-09-14 23:58:52,LilacLight,Col 69 Sector GG-X d1-3,"569,71875",-317,"-1536,15625",Col 69 Sector JG-W c2-0,"576,5625","-303,5","-1541,78125","16,1",N
+2022-09-15 01:21:47,Omega Kiowa,Pleiades Sector DL-Y d51,-101,"-153,71875","-352,53125",Pleiades Sector DL-Y d64,"-69,90625","-113,53125","-365,375","52,4",N
+2022-09-15 01:24:27,Omega Kiowa,Pleiades Sector DL-Y d64,"-69,90625","-113,53125","-365,375",Pleiades Sector CW-U b3-3,"-50,5625","-63,53125","-362,5","53,7",N
+2022-09-15 03:26:32,VIaharo,Pleiades Sector IH-V c2-5,"-78,71875","-138,03125","-321,78125",Merope,"-78,59375","-149,625","-340,53125",22,N
+2022-09-15 06:31:17,CP_Luiz,Oochost DM-P c8-0,"-421,375","-183,59375","-1982,75",Oochost DW-T d4-7,"-410,9375","-184,3125","-1919,03125","64,6",N
+2022-09-15 06:48:58,CP_Luiz,Oochost DM-P c8-0,"-421,375","-183,59375","-1982,75",Oochost DW-T d4-7,"-410,9375","-184,3125","-1919,03125","64,6",N
+2022-09-15 06:55:14,SurpriseSuprise,Aries Dark Region GM-V c2-5,"-138,5625","-121,1875","-259,875",Pleiades Sector TU-O b6-0,"-120,125","-142,03125",-304,"52,2",N
+2022-09-15 14:30:48,Sindri Völuspa,California Sector GH-L b8-0,"-279,65625","-199,75","-841,8125",California Sector JH-V c2-11,"-312,875","-217,3125","-891,09375",62,N
+2022-09-15 14:40:53,Sindri Völuspa,California Sector JH-V c2-11,"-312,875","-217,3125","-891,09375",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375","4,7",N
+2022-09-15 15:28:00,Mallchad,Pleiades Sector HR-W d1-79,"-80,625","-146,65625","-343,25",Pleiades Sector HR-W d1-65,"-85,28125",-145,"-339,40625","6,3",N
+2022-09-15 21:03:50,Mallchad,Pleiades Sector HR-W d1-17,"-84,875","-123,8125","-315,25",Pleiades Sector SZ-O b6-4,"-90,0625","-120,53125","-294,1875","21,9",N
+2022-09-15 21:04:23,LilacLight,Slegi BC-B d14-1,"-1839,09375","-629,34375","-2424,96875",Slegi DS-E b56-0,"-1844,25","-615,59375","-2420,65625","15,3",N
+2022-09-15 21:57:31,Cordon Bleu,Delphi,"-63,59375","-147,40625","-319,09375",Pleiades Sector RO-Q b5-2,"-67,03125","-136,5625","-308,09375","15,8",N
+2022-09-15 22:05:52,Cordon Bleu,Pleiades Sector TZ-O b6-2,"-78,40625","-109,09375","-294,78125",Pleiades Sector VK-N b7-1,"-78,34375","-104,59375","-284,5","11,2",N
+2022-09-15 22:42:43,Bannini,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector OS-T b3-0,"-49,65625","-167,4375","-359,5625","46,5",N
+2022-09-15 23:03:40,SurpriseSuprise,Pleiades Sector TU-O b6-0,"-120,125","-142,03125",-304,Celaeno,"-81,09375","-148,3125","-337,09375","51,6",N
+2022-09-15 23:21:37,zmouliche,Pleiades Sector ER-U b3-4,"-47,375","-83,03125","-359,875",HIP 22460,"-41,3125","-58,96875","-354,78125","25,3",Y
+2022-09-15 23:39:41,zmouliche,Col 285 Sector SB-S a6-0,"-22,3125","-42,1875","-284,03125",Synuefe VG-A b34-4,-39,"-49,9375","-335,9375","55,1",N
+2022-09-16 01:03:06,TheMightySardine,Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875",Asterope,"-80,15625","-144,09375","-333,375","22,8",N
+2022-09-16 04:10:40,CP_Luiz,Taurus Dark Region WU-O b6-1,"-40,28125","-65,75","-385,90625",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",35,Y
+2022-09-16 04:51:45,Asss,Col 69 Sector NM-U c3-1,"580,84375","-302,75",-1494,Col 69 Sector JG-W c2-0,"576,5625","-303,5","-1541,78125",48,N
+2022-09-16 07:38:24,SurpriseSuprise,Aries Dark Region GM-V c2-5,"-138,5625","-121,1875","-259,875",Pleiades Sector TU-O b6-0,"-120,125","-142,03125",-304,"52,2",N
+2022-09-16 08:08:52,CP_Luiz,Synuefe UG-A b34-0,"-48,84375","-51,1875","-331,78125",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","22,5",Y
+2022-09-16 11:53:24,Hans Acker,Pleiades Sector SE-P b6-1,"-63,90625","-103,1875","-296,78125",Pleiades Sector KC-U b3-2,"-45,46875","-140,3125","-353,6875","70,4",N
+2022-09-16 12:35:35,LilacLight,HIP 22375,"-66,90625","-66,75","-454,25",Taurus Dark Region TZ-O b6-4,"-53,75","-49,65625","-387,3125","70,3",N
+2022-09-16 15:54:59,Xanrae,Pleiades Sector AL-W b2-2,"-58,6875","-71,53125","-374,40625",HIP 22460,"-41,3125","-58,96875","-354,78125","29,1",Y
+2022-09-16 17:25:34,SmithBob,HIP 22460,"-41,3125","-58,96875","-354,78125",Taurus Dark Region XF-N b7-3,"-51,9375","-48,8125","-374,03125","24,2",Y
+2022-09-16 18:59:07,Delmonte,Col 285 Sector RB-S a6-1,"-30,875","-44,65625","-284,96875",HIP 22460,"-41,3125","-58,96875","-354,78125",72,N
+2022-09-16 19:32:55,Bommy,HIP 18908,"-138,4375","-80,6875","-390,9375",Pleiades Sector JD-R b5-3,"-113,5625","-84,5","-322,21875","73,2",N
+2022-09-16 19:57:46,HaLfY47,Hyades Sector ZU-Y c7,"-86,46875","-87,09375","-231,1875",Pleiades Sector SZ-O b6-4,"-90,0625","-120,53125","-294,1875","71,4",N
+2022-09-16 20:43:31,Lydia of the Void,Pleiades Sector GW-W d1-33,"-50,25","-62,8125","-326,8125",Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875","34,7",N
+2022-09-16 20:47:57,Alfredo Cavatelli,California Sector GW-W c1-6,"-348,125","-227,28125","-920,09375",California Sector HR-W d1-28,"-329,53125","-218,5","-893,71875","33,4",N
+2022-09-16 20:52:29,Lydia of the Void,Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875",HIP 22410,"-49,46875","-55,25","-360,59375","5,6",Y
+2022-09-16 21:39:02,Gaesatae,Merope,"-78,59375","-149,625","-340,53125",Celaeno,"-81,09375","-148,3125","-337,09375","4,4",N
+2022-09-16 21:52:59,Malcotch,California Sector KH-V c2-4,"-290,125","-211,875","-872,8125",California Sector YU-O b6-0,"-281,90625","-203,4375","-881,875","14,9",N
+2022-09-16 22:39:03,TheMightySardine,HIP 17704,"-72,03125","-133,90625","-311,09375",Asterope,"-80,15625","-144,09375","-333,375","25,8",N
+2022-09-16 23:18:45,TheMightySardine,Pleiades Sector HR-W d1-79,"-80,625","-146,65625","-343,25",Asterope,"-80,15625","-144,09375","-333,375","10,2",N
+2022-09-16 23:24:49,LilacLight,Col 69 Sector KB-W c2-0,"547,625","-316,0625","-1506,8125",Col 69 Sector KB-W c2-1,"548,625","-312,6875","-1507,5","3,6",Y
+2022-09-16 23:27:08,LilacLight,Col 69 Sector KB-W c2-1,"548,625","-312,6875","-1507,5",Col 69 Sector KB-W c2-0,"547,625","-316,0625","-1506,8125","3,6",N
+2022-09-17 00:59:37,Jimbot70,Col 69 Sector JG-W c2-0,"576,5625","-303,5","-1541,78125",Col 69 Sector KB-W c2-0,"547,625","-316,0625","-1506,8125","47,1",N
+2022-09-17 02:09:55,Jimbot70,Col 69 Sector JG-W c2-0,"576,5625","-303,5","-1541,78125",Col 69 Sector KB-W c2-0,"547,625","-316,0625","-1506,8125","47,1",N
+2022-09-17 02:18:14,Jimbot70,Col 69 Sector JG-W c2-0,"576,5625","-303,5","-1541,78125",Col 69 Sector KB-W c2-0,"547,625","-316,0625","-1506,8125","47,1",N
+2022-09-17 03:09:24,S3ARAT,HIP 18833,"-45,09375","-114,9375","-284,75",HIP 17692,"-74,125","-143,96875","-328,78125","60,2",N
+2022-09-17 04:47:48,lebonk,Musca Dark Region MD-R b5-8,"439,03125","13,21875","272,40625",Musca Dark Region PJ-P b6-1,"432,625","2,53125","288,6875","20,5",N
+2022-09-17 05:49:55,Phill P,Pleiades Sector FR-U b3-4,"-27,59375","-78,21875","-349,96875",Pleiades Sector GW-W d1-33,"-50,25","-62,8125","-326,8125","35,9",
+2022-09-17 09:18:19,Stukjes,Slegi AX-E b56-0,-1865,"-601,40625","-2419,15625",Slegi DS-E b56-0,"-1844,25","-615,59375","-2420,65625","25,2",N
+2022-09-17 09:29:13,Stukjes,Slegi AX-E b56-0,-1865,"-601,40625","-2419,15625",Slegi DS-E b56-0,"-1844,25","-615,59375","-2420,65625","25,2",N
+2022-09-17 09:32:31,Stukjes,Slegi AX-E b56-0,-1865,"-601,40625","-2419,15625",Slegi DS-E b56-0,"-1844,25","-615,59375","-2420,65625","25,2",N
+2022-09-17 09:35:04,Sindri Völuspa,Col 69 Sector KB-W c2-0,"547,625","-316,0625","-1506,8125",Col 69 Sector KB-W c2-1,"548,625","-312,6875","-1507,5","3,6",Y
+2022-09-17 09:36:13,Stukjes,Slegi AX-E b56-0,-1865,"-601,40625","-2419,15625",Slegi DS-E b56-0,"-1844,25","-615,59375","-2420,65625","25,2",N
+2022-09-17 10:44:51,Lydia of the Void,Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875",HIP 22460,"-41,3125","-58,96875","-354,78125","8,4",Y
+2022-09-17 11:00:29,Will keaton,Pleiades Sector WU-O b6-2,"-47,59375","-140,40625","-285,78125",HIP 17481,"-58,84375","-150,25","-305,53125","24,8",N
+2022-09-17 11:01:48,LilacLight,Col 69 Sector IG-W c2-0,"537,28125","-301,40625","-1542,0625",Col 69 Sector KB-W c2-0,"547,625","-316,0625","-1506,8125","39,6",N
+2022-09-17 11:10:03,Will keaton,HIP 17481,"-58,84375","-150,25","-305,53125",HR 1185,"-64,65625","-148,9375","-330,40625","25,6",N
+2022-09-17 11:22:04,Lydia of the Void,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875","8,4",Y
+2022-09-17 11:53:02,Oakwood,Col 69 Sector IG-W c2-0,"537,28125","-301,40625","-1542,0625",Col 69 Sector KB-W c2-1,"548,625","-312,6875","-1507,5","38,1",N
+2022-09-17 13:35:11,CodexNecro81,Col 69 Sector KB-W c2-0,"547,625","-316,0625","-1506,8125",Col 69 Sector KB-W c2-1,"548,625","-312,6875","-1507,5","3,6",Y
+2022-09-17 13:42:37,CHDEN49,Asterope,"-80,15625","-144,09375","-333,375",Merope,"-78,59375","-149,625","-340,53125","9,2",N
+2022-09-17 14:08:55,CHDEN49,Witch Head Sector WK-O b6-0,"299,75","-424,875","-681,25",Witch Head Sector DL-Y d8,"351,75","-419,65625","-706,5",58,N
+2022-09-17 15:26:02,Eagle131,Col 69 Sector MM-U c3-0,"538,125","-302,84375","-1500,0625",Col 69 Sector KB-W c2-0,"547,625","-316,0625","-1506,8125","17,6",N
+2022-09-17 16:35:32,Loustique,Synuefe WG-A b34-5,"-14,5","-51,15625","-329,9375",HIP 22460,"-41,3125","-58,96875","-354,78125","37,4",N
+2022-09-17 18:54:24,LilacLight,Oochost IC-S d5-4,"-375,28125","-177,4375","-1838,03125",Oochost DW-T d4-7,"-410,9375","-184,3125","-1919,03125","88,8",N
+2022-09-17 19:16:57,Mass 1nhibited,Coalsack Sector VU-O b6-6,"418,65625","24,25","304,5625",Musca Dark Region IM-V c2-24,"458,25","-8,8125","287,09375","54,5",N
+2022-09-17 19:24:14,LilacLight,Oochost IC-S d5-4,"-375,28125","-177,4375","-1838,03125",Oochost IC-S d5-3,"-365,0625","-184,28125","-1842,9375","13,2",Y
+2022-09-17 19:27:05,Mass 1nhibited,Musca Dark Region IM-V c2-24,"458,25","-8,8125","287,09375",Musca Dark Region PJ-P b6-1,"432,625","2,53125","288,6875","28,1",N
+2022-09-17 19:28:02,LilacLight,Oochost IC-S d5-3,"-365,0625","-184,28125","-1842,9375",Oochost IC-S d5-4,"-375,28125","-177,4375","-1838,03125","13,2",N
+2022-09-17 19:32:30,Ardos,Delphi,"-63,59375","-147,40625","-319,09375",Pleiades Sector IR-W d1-56,"-51,71875","-133,0625","-268,21875","54,2",
+2022-09-17 19:39:48,MossVandal,Taurus Dark Region BX-H a11-0,"-45,03125","-44,65625","-415,1875",Synuefe DQ-E a66-4,-32,"-29,8125","-359,09375","59,5",N
+2022-09-17 19:48:58,Manu Wörkl,Pleiades Sector BL-W b2-3,"-31,0625","-84,78125","-371,53125",HR 1554,"-32,375","-57,53125","-340,65625","41,2",Y
+2022-09-17 21:14:11,lebonk,Musca Dark Region PJ-P b6-1,"432,625","2,53125","288,6875",Musca Dark Region GM-V c2-35,396,"-1,90625","267,1875","42,7",N
+2022-09-17 21:46:54,Slipi,Pleiades Sector TZ-O b6-0,"-80,40625","-124,125","-304,125",Pleiades Sector HR-W d1-71,"-82,15625","-113,9375","-288,125",19,N
+2022-09-17 22:47:39,Lydia of the Void,HIP 22460,"-41,3125","-58,96875","-354,78125",HIP 22410,"-49,46875","-55,25","-360,59375","10,7",N
+2022-09-17 23:47:19,Stronzini,Horsehead Sector JC-M b7-0,"398,4375","-359,375","-763,5",Witch Head Sector EB-X c1-8,"367,375","-379,1875","-734,9375","46,6",N
+2022-09-18 00:09:44,LilacLight,Col 69 Sector PS-S c4-1,"528,0625","-282,09375","-1459,21875",Col 69 Sector LM-U c3-1,"530,8125","-290,21875","-1471,59375","15,1",N
+2022-09-18 00:34:50,LilacLight,Col 69 Sector LM-U c3-1,"530,8125","-290,21875","-1471,59375",Col 69 Sector PS-S c4-1,"528,0625","-282,09375","-1459,21875","15,1",Y
+2022-09-18 00:37:54,LilacLight,Col 69 Sector LM-U c3-1,"530,8125","-290,21875","-1471,59375",Col 69 Sector PS-S c4-1,"528,0625","-282,09375","-1459,21875","15,1",Y
+2022-09-18 00:52:00,LilacLight,Col 69 Sector PS-S c4-1,"528,0625","-282,09375","-1459,21875",Col 69 Sector LM-U c3-1,"530,8125","-290,21875","-1471,59375","15,1",N
+2022-09-18 01:01:01,LilacLight,Col 69 Sector PS-S c4-1,"528,0625","-282,09375","-1459,21875",Col 69 Sector LM-U c3-1,"530,8125","-290,21875","-1471,59375","15,1",N
+2022-09-18 01:44:39,Phill P,Col 285 Sector IV-W b2-1,"-22,53125","-60,71875","-288,875",Pleiades Sector GW-W d1-33,"-50,25","-62,8125","-326,8125",47,
+2022-09-18 01:59:32,Space_Trash,HIP 19365,"-54,8125","-69,625","-238,09375",Pleiades Sector WK-N b7-2,"-62,65625","-101,84375","-280,6875",54,N
+2022-09-18 02:19:58,Hunter_Ttc,Pleiades Sector HR-W d1-79,"-80,625","-146,65625","-343,25",Pleiades Sector IH-V c2-5,"-78,71875","-138,03125","-321,78125","23,2",N
+2022-09-18 02:24:20,Hunter_Ttc,Pleiades Sector TZ-O b6-0,"-80,40625","-124,125","-304,125",Pleiades Sector HR-W d1-71,"-82,15625","-113,9375","-288,125",19,N
+2022-09-18 02:27:13,Hunter_Ttc,Pleiades Sector HR-W d1-71,"-82,15625","-113,9375","-288,125",Pleiades Sector FW-W d1-54,"-82,875","-103,0625","-274,125","17,7",N
+2022-09-18 03:05:34,Mass 1nhibited,Wellington,"386,15625","-390,75","-692,53125",Yuanjia,"387,9375","-387,6875","-695,4375","4,6",N
+2022-09-18 03:44:06,Mass 1nhibited,Caister,"378,875","-381,4375","-721,875",Jaralis,"370,46875",-382,"-710,4375","14,2",N
+2022-09-18 04:02:01,Mass 1nhibited,Siniang,"354,40625","-386,625","-709,21875",Shenve,"351,96875","-373,46875","-711,09375","13,5",N
+2022-09-18 06:43:44,SurpriseSuprise,Aries Dark Region GM-V c2-5,"-138,5625","-121,1875","-259,875",Pleiades Sector TU-O b6-0,"-120,125","-142,03125",-304,"52,2",N
+2022-09-18 09:49:38,Lydia of the Void,HIP 22460,"-41,3125","-58,96875","-354,78125",HIP 22410,"-49,46875","-55,25","-360,59375","10,7",N
+2022-09-18 10:42:31,Surcouf87,42 n Persei,"-83,5625","-73,40625","-244,34375",Pleiades Sector SZ-O b6-4,"-90,0625","-120,53125","-294,1875","68,9",N
+2022-09-18 11:25:05,Lambast Mercy,Aries Dark Region IM-V c2-9,"-31,90625","-117,15625","-256,40625",HIP 17704,"-72,03125","-133,90625","-311,09375","69,9",N
+2022-09-18 12:17:49,Lydia of the Void,Delphi,"-63,59375","-147,40625","-319,09375",Atlas,"-76,71875","-147,34375","-344,4375","28,5",N
+2022-09-18 13:09:36,Lydia of the Void,HIP 22410,"-49,46875","-55,25","-360,59375",HIP 22460,"-41,3125","-58,96875","-354,78125","10,7",N
+2022-09-18 14:06:40,Stukjes,Col 69 Sector LM-U c3-0,"523,375","-298,5625",-1498,Col 69 Sector MM-U c3-1,538,"-281,9375","-1488,78125",24,N
+2022-09-18 14:09:59,Stukjes,Col 69 Sector MM-U c3-1,538,"-281,9375","-1488,78125",Col 69 Sector LM-U c3-1,"530,8125","-290,21875","-1471,59375","20,4",N
+2022-09-18 14:43:15,Manu Wörkl,HR 1554,"-32,375","-57,53125","-340,65625",HIP 22460,"-41,3125","-58,96875","-354,78125","16,8",Y
+2022-09-18 16:21:18,Malcotch,California Sector CV-Y c1,"-302,53125","-215,0625","-979,375",California Sector GB-X c1-4,"-300,25","-223,78125","-934,03125","46,2",N
+2022-09-18 16:21:50,Neural_Fault,Pleiades Sector GC-L b8-2,"-53,40625","-159,71875","-259,53125",HIP 17481,"-58,84375","-150,25","-305,53125","47,3",N
+2022-09-18 18:53:57,Spaxter777,Col 285 Sector AD-Z a2-3,"-40,40625","-43,5","-320,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","37,6",Y
+2022-09-18 19:42:14,StarBeaver,Pleiades Sector OO-P b6-4,"-60,34375","-64,375","-296,5625",HIP 22460,"-41,3125","-58,96875","-354,78125","61,5",N
+2022-09-18 21:17:36,Unknown Zombie,Col 69 Sector KB-W c2-0,"547,625","-316,0625","-1506,8125",Col 69 Sector MM-U c3-1,538,"-281,9375","-1488,78125","39,8",N
+2022-09-18 21:24:12,Unknown Zombie,Col 69 Sector MM-U c3-1,538,"-281,9375","-1488,78125",Col 69 Sector LM-U c3-1,"530,8125","-290,21875","-1471,59375","20,4",N
+2022-09-19 00:13:59,Sinister Hedgehog,Hyades Sector JS-Z a1-0,"-50,0625","-27,0625","-262,03125",Col 285 Sector UW-A a2-3,"-59,59375","-37,125","-325,6875","65,1",N
+2022-09-19 01:43:33,Coldwillow,Pleiades Sector XE-Y b1-0,"-39,59375","-82,65625",-400,HIP 22460,"-41,3125","-58,96875","-354,78125","51,1",N
+2022-09-19 02:15:14,Will keaton,Asterope,"-80,15625","-144,09375","-333,375",Merope,"-78,59375","-149,625","-340,53125","9,2",N
+2022-09-19 02:22:31,LegendaryHobo,Witch Head Sector KC-V c2-3,"355,6875","-399,5","-700,65625",Ronemar,"355,75","-400,5","-707,21875","6,6",N
+2022-09-19 03:25:51,Kretak,Taurus Dark Region XF-N b7-1,"-49,78125","-48,625","-366,4375",HIP 22460,"-41,3125","-58,96875","-354,78125","17,7",Y
+2022-09-19 06:40:34,Falcon-Screech,Pleiades Sector VU-O b6-0,"-75,0625","-127,65625","-286,96875",Pleiades Sector GW-W c1-9,"-94,375","-151,03125","-354,15625","73,7",N
+2022-09-19 09:00:06,LilacLight,HD 38659,"532,8125","-287,90625","-1422,21875",Col 69 Sector LM-U c3-1,"530,8125","-290,21875","-1471,59375","49,5",N
+2022-09-19 09:06:17,Neutronic1,Asterope,"-80,15625","-144,09375","-333,375",HIP 17692,"-74,125","-143,96875","-328,78125","7,6",N
+2022-09-19 14:11:38,Jo Quinn,Pleiades Sector NI-S b4-3,"-76,40625","-131,875","-335,9375",Pleiades Sector GW-W c1-9,"-94,375","-151,03125","-354,15625",32,N
+2022-09-19 17:28:45,LilacLight,Slegi AH-B d14-1,"-1806,25","-563,9375","-2416,0625",Slegi DS-E b56-0,"-1844,25","-615,59375","-2420,65625","64,3",N
+2022-09-19 21:03:54,Mass 1nhibited,California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","45,3",N
+2022-09-19 21:15:15,LilacLight,Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875",Slegi CC-B d14-1,-1766,"-593,9375","-2366,75","14,8",Y
+2022-09-19 21:17:34,LilacLight,Slegi CC-B d14-1,-1766,"-593,9375","-2366,75",Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875","14,8",N
+2022-09-19 21:28:39,Mass 1nhibited,California Sector HR-W d1-28,"-329,53125","-218,5","-893,71875",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375","15,9",N
+2022-09-19 21:30:06,RottenPineapple,Col 69 Sector PS-S c4-1,"528,0625","-282,09375","-1459,21875",Col 69 Sector LM-U c3-1,"530,8125","-290,21875","-1471,59375","15,1",N
+2022-09-19 21:43:14,Wheity,Slegi OE-B b58-0,"-1781,03125","-621,59375","-2379,6875",Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875","32,7",N
+2022-09-19 22:13:18,Jeff slater,Synuefe AN-Y b34-0,-22,"-61,6875","-322,375",HIP 22460,"-41,3125","-58,96875","-354,78125","37,8",
+2022-09-19 23:31:54,quinnrz,Slegi QP-Z b58-0,"-1770,90625","-602,0625","-2358,21875",Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875","15,5",N
+2022-09-20 00:01:04,quinnrz,Slegi MJ-B b58-0,"-1784,4375","-586,25","-2379,71875",Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875","16,1",N
+2022-09-20 01:01:25,quinnrz,Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875",Slegi CC-B d14-1,-1766,"-593,9375","-2366,75","14,8",N
+2022-09-20 01:30:26,Kretak,Synuefe NX-A a68-1,"-26,09375","-38,03125","-340,4375",HIP 22711,"-35,75","-60,84375","-366,90625","36,3",Y
+2022-09-20 06:08:11,Peter Drakon,Slegi MJ-B b58-0,"-1784,4375","-586,25","-2379,71875",Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875","16,1",N
+2022-09-20 08:01:34,matheusgames,HIP 22711,"-35,75","-60,84375","-366,90625",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","17,8",
+2022-09-20 08:05:07,matheusgames,HR 1554,"-32,375","-57,53125","-340,65625",Synuefe GX-B c16-5,"-32,9375","-57,5625","-348,15625","7,5",
+2022-09-20 08:11:56,matheusgames,Synuefe GX-B c16-5,"-32,9375","-57,5625","-348,15625",HIP 22711,"-35,75","-60,84375","-366,90625","19,2",
+2022-09-20 14:39:08,Imviral,Maia,"-81,78125","-149,4375","-343,375",Celaeno,"-81,09375","-148,3125","-337,09375","6,4",N
+2022-09-20 18:06:25,LilacLight,Col 69 Sector UY-Q c5-12,"540,78125","-288,21875","-1421,53125",HD 38659,"532,8125","-287,90625","-1422,21875",8,N
+2022-09-20 18:19:17,msTheo,Pleiades Sector DB-X c1-5,"-106,9375","-138,25","-380,40625",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","52,2",N
+2022-09-20 18:21:06,Stukjes,Col 69 Sector PS-S c4-3,"526,9375","-296,78125","-1429,53125",Col 69 Sector PS-S c4-7,"528,5","-274,71875","-1428,0625","22,2",N
+2022-09-20 20:41:20,Malcotch,California Sector DL-Y d18,"-322,40625",-257,"-922,75",California Sector SI-T b3-0,"-322,125","-274,15625","-926,96875","17,7",N
+2022-09-20 21:26:18,teeter jenkins,HIP 29630,"157,5","-15,40625","-485,28125",Taurus Dark Region YF-N b7-3,"-28,96875","-55,375","-366,1875","224,8",N
+2022-09-20 22:03:10,Sarusoid,Hyades Sector YJ-Z b3,"-18,03125","-65,40625","-254,53125",Pleiades Sector SE-P b6-4,"-61,90625","-94,21875","-298,59375","68,5",
+2022-09-20 22:12:58,Firehawk894,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","4,4",
+2022-09-20 22:25:56,Firehawk894,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","4,4",
+2022-09-20 22:26:48,Firehawk894,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","4,4",
+2022-09-20 22:28:31,Firehawk894,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Asterope,"-80,15625","-144,09375","-333,375","4,4",
+2022-09-20 22:42:16,Firehawk894,Asterope,"-80,15625","-144,09375","-333,375",Celaeno,"-81,09375","-148,3125","-337,09375","5,7",
+2022-09-20 22:57:14,Asss,HIP 16374,"-56,6875","-151,9375","-263,59375",HIP 17704,"-72,03125","-133,90625","-311,09375","53,1",N
+2022-09-21 03:19:12,allan mandragoran,Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875",Pleiades Sector MI-S b4-1,"-103,375","-138,3125","-339,8125","4,8",N
+2022-09-21 03:33:09,allan mandragoran,Pleiades Sector IH-V c2-16,-92,"-143,5","-341,3125",Pleiades Sector KC-V c2-10,"-87,3125","-149,90625","-343,46875","8,2",N
+2022-09-21 04:27:25,quinnrz,Slegi CC-B d14-1,-1766,"-593,9375","-2366,75",Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875","14,8",N
+2022-09-21 08:57:02,PanPiper,California Sector IH-V c2-2,"-352,09375","-218,03125","-888,53125",California Sector SJ-Q b5-0,"-363,03125","-219,125","-900,25","16,1",N
+2022-09-21 09:12:40,PanPiper,California Sector MD-S b4-0,"-392,4375","-212,40625","-917,1875",California Sector EB-X c1-3,"-376,125","-218,125","-937,53125","26,7",N
+2022-09-21 10:38:44,PanPiper,California Sector ZZ-Y c9,-318,"-184,125","-952,4375",California Sector BV-Y c9,"-312,53125","-192,03125","-948,15625","10,5",N
+2022-09-21 10:42:27,PanPiper,California Sector BV-Y c9,"-312,53125","-192,03125","-948,15625",California Sector CQ-Y d28,"-304,3125",-183,"-948,9375","12,2",N
+2022-09-21 10:51:46,Molan Ryke,Wregoe ON-O b33-0,"-56,875","-5,6875","-342,3125",Pleiades Sector AL-X c1-7,"-71,53125","-61,375","-351,46875","58,3",N
+2022-09-21 15:12:21,quinnrz,Slegi CC-B d14-1,-1766,"-593,9375","-2366,75",Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875","14,8",N
+2022-09-21 15:15:11,quinnrz,Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875",Slegi CC-B d14-1,-1766,"-593,9375","-2366,75","14,8",Y
+2022-09-21 15:20:07,quinnrz,Slegi CC-B d14-1,-1766,"-593,9375","-2366,75",Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875","14,8",N
+2022-09-21 15:28:22,quinnrz,Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875",Slegi QP-Z b58-0,"-1770,90625","-602,0625","-2358,21875","15,5",Y
+2022-09-21 16:05:01,Hurix,Synuefe RA-C b33-0,"-25,875","-50,5","-364,125",Taurus Dark Region YF-N b7-3,"-28,96875","-55,375","-366,1875","6,1",Y
+2022-09-21 16:07:45,Hurix,Taurus Dark Region YF-N b7-3,"-28,96875","-55,375","-366,1875",HIP 22711,"-35,75","-60,84375","-366,90625","8,7",Y
+2022-09-21 16:27:40,quinnrz,Slegi MJ-B b58-0,"-1784,4375","-586,25","-2379,71875",Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875","16,1",N
+2022-09-21 16:57:55,quinnrz,Slegi MJ-B b58-0,"-1784,4375","-586,25","-2379,71875",Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875","16,1",N
+2022-09-21 17:03:02,quinnrz,Slegi MJ-B b58-0,"-1784,4375","-586,25","-2379,71875",Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875","16,1",N
+2022-09-21 17:18:06,quinnrz,Slegi CC-B d14-1,-1766,"-593,9375","-2366,75",Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875","14,8",N
+2022-09-21 17:22:37,quinnrz,Slegi CC-B d14-1,-1766,"-593,9375","-2366,75",Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875","14,8",N
+2022-09-21 19:27:27,LilacLight,Oochost FU-J b28-0,"-364,90625","-179,90625","-1740,34375",Oochost AO-L b27-0,"-365,59375","-182,46875","-1752,40625","12,4",N
+2022-09-21 19:39:25,quinnrz,Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875",Slegi CC-B d14-1,-1766,"-593,9375","-2366,75","14,8",Y
+2022-09-21 19:58:07,LilacLight,Col 69 Sector UY-Q c5-12,"540,78125","-288,21875","-1421,53125",HD 38659,"532,8125","-287,90625","-1422,21875",8,N
+2022-09-21 20:23:34,quinnrz,Slegi MJ-B b58-0,"-1784,4375","-586,25","-2379,71875",Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875","16,1",N
+2022-09-21 20:30:27,quinnrz,Slegi MJ-B b58-0,"-1784,4375","-586,25","-2379,71875",Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875","16,1",N
+2022-09-21 20:46:19,PercyNoMercy,Pleiades Sector DB-W b2-3,"-80,21875","-107,96875",-371,Pleiades Sector EG-W b2-1,"-9,5625","-97,59375","-376,125","71,6",N
+2022-09-21 20:46:27,quinnrz,Slegi CC-B d14-1,-1766,"-593,9375","-2366,75",Slegi QP-Z b58-0,"-1770,90625","-602,0625","-2358,21875","12,8",N
+2022-09-21 20:52:04,quinnrz,Slegi MJ-B b58-0,"-1784,4375","-586,25","-2379,71875",Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875","16,1",N
+2022-09-21 20:58:12,quinnrz,Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875",Slegi MJ-B b58-0,"-1784,4375","-586,25","-2379,71875","16,1",Y
+2022-09-21 22:00:05,LilacLight,Orion Dark Region CB-X c1-2,"525,5","-288,4375","-1379,59375",Orion Dark Region CB-X c1-1,"526,625","-283,15625","-1376,21875","6,4",N
+2022-09-21 22:03:35,LilacLight,Orion Dark Region CB-X c1-2,"525,5","-288,4375","-1379,59375",Orion Dark Region CB-X c1-1,"526,625","-283,15625","-1376,21875","6,4",N
+2022-09-21 22:22:39,Molan Ryke,Pleiades Sector GM-U b3-0,"-48,375","-97,09375","-351,28125",Pleiades Sector FR-U b3-0,"-38,4375",-69,"-359,96875",31,Y
+2022-09-21 22:30:00,Molan Ryke,HIP 22460,"-41,3125","-58,96875","-354,78125",HIP 22711,"-35,75","-60,84375","-366,90625","13,5",Y
+2022-09-21 22:34:32,Molan Ryke,Pleiades Sector CG-W b2-1,"-58,75","-85,5625","-370,90625",Pleiades Sector HH-U b3-2,"-66,34375","-117,28125",-364,"33,3",Y
+2022-09-21 22:43:22,Gray Z7Z,Pleiades Sector GW-W d1-50,"-44,1875","-92,34375","-279,59375",Pleiades Sector IH-V c2-5,"-78,71875","-138,03125","-321,78125","71,1",N
+2022-09-22 03:00:18,Kretak,Synuefe NX-A a68-1,"-26,09375","-38,03125","-340,4375",HIP 22711,"-35,75","-60,84375","-366,90625","36,3",Y
+2022-09-22 03:44:27,quinnrz,Slegi CC-B d14-1,-1766,"-593,9375","-2366,75",Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875","14,8",N
+2022-09-22 03:55:32,quinnrz,Slegi NJ-B b58-0,"-1764,28125","-600,1875","-2379,65625",Slegi CC-B d14-1,-1766,"-593,9375","-2366,75","14,4",N
+2022-09-22 03:58:38,quinnrz,Slegi NJ-B b58-0,"-1764,28125","-600,1875","-2379,65625",Slegi CC-B d14-1,-1766,"-593,9375","-2366,75","14,4",N
+2022-09-22 04:13:59,quinnrz,Slegi NJ-B b58-0,"-1764,28125","-600,1875","-2379,65625",Slegi CC-B d14-1,-1766,"-593,9375","-2366,75","14,4",N
+2022-09-22 04:32:37,quinnrz,Slegi CC-B d14-1,-1766,"-593,9375","-2366,75",Slegi NJ-B b58-0,"-1764,28125","-600,1875","-2379,65625","14,4",N
+2022-09-22 04:50:01,quinnrz,Slegi NJ-B b58-0,"-1764,28125","-600,1875","-2379,65625",Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875","23,2",N
+2022-09-22 10:38:13,LilacLight,Oochost NG-G b30-0,"-354,5","-181,34375","-1687,28125",Oochost AO-L b27-0,"-365,59375","-182,46875","-1752,40625","66,1",N
+2022-09-22 11:01:03,Wotherspoon,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",Y
+2022-09-22 11:04:42,LilacLight,Oochost NG-G b30-0,"-354,5","-181,34375","-1687,28125",Oochost AO-L b27-0,"-365,59375","-182,46875","-1752,40625","66,1",N
+2022-09-22 11:55:26,Ceres_O7,Pleiades Sector HR-W d1-59,"-76,03125","-128,3125","-326,25",Asterope,"-80,15625","-144,09375","-333,375","17,8",N
+2022-09-22 13:12:16,Latambadam,HIP 17862,"-81,4375","-151,90625","-359,59375",HIP 17403,"-93,6875","-158,96875","-367,625","16,3",N
+2022-09-22 13:52:53,LilacLight,Orion Dark Region CL-Y d17,"509,4375","-277,34375","-1335,78125",Orion Dark Region CB-X c1-1,"526,625","-283,15625","-1376,21875","44,3",N
+2022-09-22 14:15:02,Katie Drakon,HD 38659,"532,8125","-287,90625","-1422,21875",Orion Dark Region AB-W b2-0,"535,71875","-281,75","-1384,9375","37,9",N
+2022-09-22 14:18:32,LilacLight,Oochost XH-N b26-0,"-352,375","-178,375","-1779,875",Oochost AO-L b27-0,"-365,59375","-182,46875","-1752,40625","30,8",N
+2022-09-22 14:24:05,quinnrz,Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875",Slegi CC-B d14-1,-1766,"-593,9375","-2366,75","14,8",Y
+2022-09-22 15:13:37,quinnrz,Slegi QP-Z b58-0,"-1770,90625","-602,0625","-2358,21875",Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875","15,5",N
+2022-09-22 15:45:49,caRGOrunner1969,Pleiades Sector LN-S b4-3,"-76,1875","-119,59375","-326,15625",HR 1183,"-72,875","-145,09375","-336,96875","27,9",
+2022-09-22 15:47:24,caRGOrunner1969,Pleiades Sector LN-S b4-3,"-76,1875","-119,59375","-326,15625",HR 1183,"-72,875","-145,09375","-336,96875","27,9",
+2022-09-22 18:09:00,Space Mickk,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector VK-N b7-0,"-81,625","-100,21875","-279,375","69,6",N
+2022-09-22 18:25:06,Latambadam,HIP 17403,"-93,6875","-158,96875","-367,625",Pleiades Sector IH-V c2-14,"-88,03125","-142,21875","-338,875","33,8",N
+2022-09-22 20:06:22,PeterBob,HIP 17525,"-73,8125","-113,6875","-277,21875",Pleiades Sector MN-T c3-1,"-69,75","-131,625","-290,4375","22,6",N
+2022-09-22 20:19:25,PeterBob,HIP 17692,"-74,125","-143,96875","-328,78125",Merope,"-78,59375","-149,625","-340,53125","13,8",N
+2022-09-23 01:10:44,Ceres_O7,Pleiades Sector HH-U b3-0,"-70,4375","-121,5625","-357,8125",Pleiades Sector DG-X c1-15,"-55,09375","-101,65625","-353,375","25,5",N
+2022-09-23 01:13:38,Ceres_O7,Pleiades Sector DG-X c1-15,"-55,09375","-101,65625","-353,375",Pleiades Sector ER-U b3-2,"-52,875","-77,71875","-349,375","24,4",Y
+2022-09-23 01:57:14,Ceres_O7,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","5,2",Y
+2022-09-23 02:38:48,Ceres_O7,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",Y
+2022-09-23 03:01:27,Ceres_O7,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",Y
+2022-09-23 03:13:36,Ceres_O7,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","5,2",Y
+2022-09-23 03:17:42,LilacLight,Col 69 Sector TY-Q c5-6,"530,03125","-276,4375","-1407,5625",Orion Dark Region CB-X c1-1,"526,625","-283,15625","-1376,21875","32,2",N
+2022-09-23 11:02:05,caRGOrunner1969,Pleiades Sector IH-V c2-13,"-84,15625","-137,5",-328,Asterope,"-80,15625","-144,09375","-333,375","9,4",
+2022-09-23 12:33:26,TheMightySardine,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector IH-V c2-17,"-91,78125","-141,0625","-340,375","13,9",N
+2022-09-23 14:26:56,Luna sky,HIP 22460,"-41,3125","-58,96875","-354,78125",Synuefe KD-A c17-9,"-30,6875","-56,90625","-329,96875","27,1",Y
+2022-09-23 17:19:59,Requese,Oochoss LL-D c1,"-1762,46875","-581,84375","-2319,875",Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875","49,9",N
+2022-09-23 17:58:37,ENERGYMR,Pleiades Sector HW-W c1-10,"-33,625","-162,75","-354,375",HIP 17694,"-72,25","-152,53125","-338,6875","42,9",N
+2022-09-23 19:10:55,ENERGYMR,Pleiades Sector GW-W c1-15,"-76,9375","-153,71875","-346,625",HIP 16217,"-107,84375","-155,5625","-333,28125","33,7",N
+2022-09-23 19:46:28,LilacLight,Oochoss ZR-G b0,"-1762,46875","-601,28125","-2344,625",Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875","28,9",N
+2022-09-23 19:55:08,Avasa Siuu,Pleiades Sector QD-S b4-1,"-57,21875","-149,375","-337,125",HR 1185,"-64,65625","-148,9375","-330,40625",10,N
+2022-09-23 20:18:42,lAMOTHE,Witch Head Sector JC-U b3-0,"359,4375","-384,9375","-742,65625",Shenve,"351,96875","-373,46875","-711,09375","34,4",
+2022-09-23 20:36:06,badloop1,HIP 17403,"-93,6875","-158,96875","-367,625",Pleiades Sector HR-W d1-74,"-87,40625","-150,90625","-344,96875","24,9",N
+2022-09-23 20:39:34,badloop1,Pleiades Sector RO-Q b5-0,"-80,46875","-140,21875","-324,15625",Pleiades Sector RO-Q b5-3,"-70,46875","-131,8125","-306,78125","21,7",N
+2022-09-23 20:55:57,ENERGYMR,Delphi,"-63,59375","-147,40625","-319,09375",HIP 17694,"-72,25","-152,53125","-338,6875",22,N
+2022-09-23 21:35:10,Avasa Siuu,HR 1185,"-64,65625","-148,9375","-330,40625",Asterope,"-80,15625","-144,09375","-333,375","16,5",N
+2022-09-23 21:56:30,Wheity,Oochoss ZR-G b0,"-1762,46875","-601,28125","-2344,625",Slegi CC-B d14-4,"-1780,65625","-592,21875","-2365,21875","28,9",N
+2022-09-23 22:49:11,Firehawk894,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",Asterope,"-80,15625","-144,09375","-333,375","9,2",
+2022-09-23 23:11:37,Tianve Lave,HIP 22334,"-24,03125","-85,5","-393,78125",Pleiades Sector DG-X c1-14,"-45,625","-80,78125","-376,4375","28,1",N
+2022-09-24 00:14:15,Istarias,Celaeno,"-81,09375","-148,3125","-337,09375",Asterope,"-80,15625","-144,09375","-333,375","5,7",N
+2022-09-24 02:32:36,Denis Page,Taurus Dark Region HJ-E a13-2,"-66,40625","-44,59375","-398,15625",Taurus Dark Region XF-N b7-1,"-49,78125","-48,625","-366,4375",36,N
+2022-09-24 04:17:05,quinnrz,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Asterope,"-80,15625","-144,09375","-333,375","4,4",N
+2022-09-24 04:26:54,Sageking098,HIP 17692,"-74,125","-143,96875","-328,78125",Pleiades Sector NN-T c3-9,"-43,21875","-130,25","-265,15625","72,1",N
+2022-09-24 06:14:12,allan mandragoran,Pleiades Sector IH-V c2-15,"-87,34375","-144,96875","-344,25",Merope,"-78,59375","-149,625","-340,53125","10,6",N
+2022-09-24 08:32:43,Ceres_O7,Pleiades Sector CG-X c1-12,"-65,0625","-101,03125","-348,53125",Pleiades Sector HH-U b3-0,"-70,4375","-121,5625","-357,8125","23,2",Y
+2022-09-24 11:31:57,TheMightySardine,Asterope,"-80,15625","-144,09375","-333,375",Celaeno,"-81,09375","-148,3125","-337,09375","5,7",N
+2022-09-24 11:42:58,TheMightySardine,Celaeno,"-81,09375","-148,3125","-337,09375",Asterope,"-80,15625","-144,09375","-333,375","5,7",N
+2022-09-24 12:02:16,TheMightySardine,Celaeno,"-81,09375","-148,3125","-337,09375",Asterope,"-80,15625","-144,09375","-333,375","5,7",N
+2022-09-24 12:13:52,SturmWaffel,Pleiades Sector SZ-O b6-3,"-94,8125","-118,5","-296,34375",Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875","51,7",N
+2022-09-24 12:35:12,TheMightySardine,Celaeno,"-81,09375","-148,3125","-337,09375",Asterope,"-80,15625","-144,09375","-333,375","5,7",N
+2022-09-24 12:39:33,TheMightySardine,Celaeno,"-81,09375","-148,3125","-337,09375",Asterope,"-80,15625","-144,09375","-333,375","5,7",N
+2022-09-24 16:25:28,ENERGYMR,Pleiades Sector HR-W d1-57,"-86,40625","-125,15625","-301,84375",Pleiades Sector EB-X c1-18,"-87,84375","-142,75","-350,96875","52,2",N
+2022-09-24 17:44:40,Yeri-san,HIP 17125,"-95,875","-128,75","-316,5625",Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875","29,1",N
+2022-09-25 11:15:49,MattW,HIP 17403,"-93,6875","-158,96875","-367,625",Pleiades Sector IR-W d1-39,"-50,21875","-139,46875","-335,96875","57,2",N
+2022-09-25 12:50:31,CodexNecro81,Oochost IF-I b29-0,"-340,46875","-159,03125","-1720,375",Oochost AO-L b27-0,"-365,59375","-182,46875","-1752,40625",47,N
+2022-09-25 13:06:28,EREBUS5,Oochost FU-J b28-0,"-364,90625","-179,90625","-1740,34375",Oochost AO-L b27-0,"-365,59375","-182,46875","-1752,40625","12,4",N
+2022-09-25 13:50:26,TomCatT,HIP 17704,"-72,03125","-133,90625","-311,09375",HR 1183,"-72,875","-145,09375","-336,96875","28,2",N
+2022-09-25 13:55:54,TheMightySardine,Celaeno,"-81,09375","-148,3125","-337,09375",Asterope,"-80,15625","-144,09375","-333,375","5,7",N
+2022-09-25 14:08:37,TheMightySardine,Celaeno,"-81,09375","-148,3125","-337,09375",Asterope,"-80,15625","-144,09375","-333,375","5,7",N
+2022-09-25 14:26:07,TheMightySardine,Celaeno,"-81,09375","-148,3125","-337,09375",Asterope,"-80,15625","-144,09375","-333,375","5,7",N
+2022-09-25 14:35:18,StarBeaver,Asterope,"-80,15625","-144,09375","-333,375",HR 1183,"-72,875","-145,09375","-336,96875","8,2",N
+2022-09-25 14:38:52,TheMightySardine,Celaeno,"-81,09375","-148,3125","-337,09375",Asterope,"-80,15625","-144,09375","-333,375","5,7",N
+2022-09-25 14:44:25,PanPiper,HD 26080,"-318,46875","-193,25","-973,21875",California Sector GW-V b2-0,"-324,34375","-199,9375","-962,46875",14,N
+2022-09-25 14:47:53,TheMightySardine,Celaeno,"-81,09375","-148,3125","-337,09375",Asterope,"-80,15625","-144,09375","-333,375","5,7",N
+2022-09-25 14:51:40,TheMightySardine,Asterope,"-80,15625","-144,09375","-333,375",Celaeno,"-81,09375","-148,3125","-337,09375","5,7",N
+2022-09-25 15:03:38,TheMightySardine,Asterope,"-80,15625","-144,09375","-333,375",Celaeno,"-81,09375","-148,3125","-337,09375","5,7",N
+2022-09-25 15:10:35,StarBeaver,HR 1183,"-72,875","-145,09375","-336,96875",Asterope,"-80,15625","-144,09375","-333,375","8,2",N
+2022-09-25 15:31:50,PanPiper,California Sector AV-Y c4,"-365,5","-217,96875","-952,53125",California Sector KX-T b3-0,"-362,84375","-219,65625","-942,1875","10,8",N
+2022-09-25 15:32:10,Malcotch,California Sector GB-X c1-4,"-300,25","-223,78125","-934,03125",California Sector PS-T b3-0,"-297,90625","-236,71875","-935,59375","13,2",N
+2022-09-25 15:35:29,PanPiper,California Sector KX-T b3-0,"-362,84375","-219,65625","-942,1875",California Sector IC-U b3-0,"-361,625","-199,625","-940,84375","20,1",N
+2022-09-25 20:14:59,Malcotch,HIP 18458,"-305,375","-242,15625","-914,875",California Sector MC-V c2-9,"-301,5625","-232,875","-897,53125",20,N
+2022-09-25 20:21:53,HunabKU,Col 285 Sector NV-T a5-0,"-34,53125","-42,625","-294,84375",Col 285 Sector DP-Y b1-5,"-38,03125","-48,71875","-320,9375",27,N
+2022-09-25 20:25:20,pokegustavo,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",Y
+2022-09-25 20:28:43,HunabKU,Synuefe GX-B c16-6,"-37,25","-55,125","-347,15625",HIP 22460,"-41,3125","-58,96875","-354,78125","9,5",Y
+2022-09-25 20:31:30,HunabKU,Synuefe GX-B c16-6,"-37,25","-55,125","-347,15625",HIP 22460,"-41,3125","-58,96875","-354,78125","9,5",Y
+2022-09-25 22:08:17,apsidalcolt,Onoros,"389,71875","-379,9375","-724,15625",Witch Head Sector OT-Q b5-0,"337,625","-359,71875","-701,125","60,4",N
+2022-09-26 00:52:55,Gray Z7Z,Taygeta,"-88,5","-159,6875","-366,28125",Sterope II,"-81,65625","-147,28125","-340,84375","29,1",N
+2022-09-26 00:58:00,Gray Z7Z,Sterope II,"-81,65625","-147,28125","-340,84375",Delphi,"-63,59375","-147,40625","-319,09375","28,3",N
+2022-09-26 01:29:21,Gray Z7Z,Delphi,"-63,59375","-147,40625","-319,09375",Pleiades Sector HR-W d1-42,"-70,1875","-150,21875","-343,84375","25,8",N
+2022-09-26 01:35:18,Gray Z7Z,Pleiades Sector HR-W d1-42,"-70,1875","-150,21875","-343,84375",Sterope II,"-81,65625","-147,28125","-340,84375","12,2",N
+2022-09-26 01:59:20,Ceres_O7,Pleiades Sector TZ-O b6-3,"-74,375","-115,71875","-294,53125",Pleiades Sector HR-W d1-79,"-80,625","-146,65625","-343,25",58,N
+2022-09-26 02:59:28,ReiDusGelo,Coalsack Sector KN-S b4-6,"410,75","35,6875","263,03125",Coalsack Sector KN-S b4-9,"405,46875",40,"264,0625","6,9",N
+2022-09-26 03:13:57,Ceres_O7,Maia,"-81,78125","-149,4375","-343,375",Pleiades Sector NI-S b4-2,"-66,4375","-131,375","-336,21875","24,8",N
+2022-09-26 03:18:13,Ceres_O7,Pleiades Sector IX-S b4-3,"-62,0625","-84,46875","-342,3125",Pleiades Sector ER-U b3-1,"-55,40625","-71,84375","-348,78125","15,7",Y
+2022-09-26 03:38:13,Ceres_O7,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",Y
+2022-09-26 03:38:17,allan mandragoran,Pleiades Sector HR-W d1-36,"-67,65625","-150,71875","-333,3125",Merope,"-78,59375","-149,625","-340,53125","13,2",N
+2022-09-26 04:06:08,Ceres_O7,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","5,2",Y
+2022-09-26 07:33:02,Ceres_O7,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","5,2",Y
+2022-09-26 16:36:13,Mass 1nhibited,California Sector AW-M b7-0,"-360,15625","-219,3125","-860,96875",California Sector EB-X c1-1,"-365,3125","-223,3125","-931,25","70,6",N
+2022-09-26 18:54:33,Yeri-san,Pleiades Sector IH-V c2-0,"-104,3125","-140,4375","-344,78125",Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875","4,4",N
+2022-09-26 19:26:37,Beetlejude,California Sector OS-T b3-0,"-322,1875","-239,3125","-941,59375",California Sector IR-W d1-23,"-302,53125","-228,5625","-888,0625",58,N
+2022-09-26 20:52:09,allan mandragoran,Merope,"-78,59375","-149,625","-340,53125",Maia,"-81,78125","-149,4375","-343,375","4,3",N
+2022-09-26 22:27:39,quinnrz,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Celaeno,"-81,09375","-148,3125","-337,09375","4,6",N
+2022-09-26 22:38:12,Gray Z7Z,Hyades Sector VD-B b3,"-1,96875","-72,59375","-271,03125",Pleiades Sector RT-Q b5-2,"-42,09375","-120,28125","-306,625","71,8",N
+2022-09-26 22:44:52,Gray Z7Z,Sterope II,"-81,65625","-147,28125","-340,84375",Delphi,"-63,59375","-147,40625","-319,09375","28,3",N
+2022-09-26 23:16:52,Gray Z7Z,Delphi,"-63,59375","-147,40625","-319,09375",Pleiades Sector HR-W d1-42,"-70,1875","-150,21875","-343,84375","25,8",N
+2022-09-27 00:44:10,Istarias,Celaeno,"-81,09375","-148,3125","-337,09375",Asterope,"-80,15625","-144,09375","-333,375","5,7",N
+2022-09-27 01:00:39,Gray Z7Z,Sterope II,"-81,65625","-147,28125","-340,84375",Taygeta,"-88,5","-159,6875","-366,28125","29,1",N
+2022-09-27 05:54:31,apsidalcolt,Pleiades Sector IG-X b1-0,"-17,3125","-182,5","-399,09375",Pleiades Sector MX-T b3-0,"-59,53125","-161,5625","-363,375","59,1",N
+2022-09-27 17:22:44,Gray Z7Z,Pleiades Sector KC-V c2-4,"-88,8125","-148,71875","-321,1875",Pleiades Sector IH-V c2-5,"-78,71875","-138,03125","-321,78125","14,7",N
+2022-09-27 18:13:06,StarBeaver,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Asterope,"-80,15625","-144,09375","-333,375","4,4",N
+2022-09-27 21:25:19,Yeri-san,Hyades Sector DB-X c1-3,"-86,15625","-86,75","-215,0625",Pleiades Sector MN-T c3-2,"-103,21875","-115,78125","-267,78125","62,6",N
+2022-09-27 23:55:54,Ashurson,HIP 18390,"-299,0625","-229,25","-876,125",California Sector GB-X c1-2,"-286,125","-208,375","-913,21875","44,5",N
+2022-09-28 00:31:59,Gray Z7Z,Pleiades Sector KC-V c2-4,"-88,8125","-148,71875","-321,1875",HIP 17694,"-72,25","-152,53125","-338,6875","24,4",N
+2022-09-28 00:54:07,Avasa Siuu,Pleiades Sector TJ-Q b5-0,"-76,09375","-147,78125","-308,4375",Asterope,"-80,15625","-144,09375","-333,375","25,5",N
+2022-09-28 01:29:08,Gray Z7Z,Pleiades Sector HR-W d1-42,"-70,1875","-150,21875","-343,84375",Sterope II,"-81,65625","-147,28125","-340,84375","12,2",N
+2022-09-28 03:33:25,MossVandal,Pleiades Sector OD-S b4-1,"-93,40625",-164,"-341,59375",HIP 17034,"-92,1875","-157,625","-346,90625","8,4",N
+2022-09-28 12:33:20,HunabKU,Pleiades Sector LN-S b4-2,"-66,75","-107,5","-333,59375",Pleiades Sector IX-S b4-1,"-60,78125","-79,53125","-344,90625","30,8",N
+2022-09-28 12:49:13,HunabKU,Pleiades Sector IX-S b4-1,"-60,78125","-79,53125","-344,90625",Pleiades Sector DG-X c1-11,"-49,25","-70,4375","-360,8125","21,6",Y
+2022-09-29 00:34:02,quinnrz,Pleiades Sector HR-W d1-17,"-84,875","-123,8125","-315,25",Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875","35,6",N
+2022-09-29 01:46:46,Secretpro,Pleione,-77,"-146,78125","-344,125",Asterope,"-80,15625","-144,09375","-333,375","11,5",N
+2022-09-29 06:12:02,Reyedog,Synuefe KD-A c17-9,"-30,6875","-56,90625","-329,96875",HIP 22460,"-41,3125","-58,96875","-354,78125","27,1",Y
+2022-09-29 07:59:23,doggie015,Musca Dark Region HX-S b4-10,"422,8125","5,4375","239,53125",HIP 62154,"411,375","9,09375","261,46875",25,N
+2022-09-29 10:09:09,Reyedog,Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875",HIP 22460,"-41,3125","-58,96875","-354,78125","8,4",Y
+2022-09-29 11:45:54,GCSpitfire,Pleiades Sector LX-U d2-43,"-84,4375","-133,34375","-254,59375",Pleiades Sector IH-V c2-11,"-89,5625","-143,125","-317,21875","63,6",N
+2022-09-29 11:49:46,GCSpitfire,Pleiades Sector IH-V c2-11,"-89,5625","-143,125","-317,21875",Merope,"-78,59375","-149,625","-340,53125","26,6",N
+2022-09-29 14:39:45,SturmWaffel,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875","22,8",N
+2022-09-29 17:04:24,Avasa Siuu,Asterope,"-80,15625","-144,09375","-333,375",HIP 17692,"-74,125","-143,96875","-328,78125","7,6",N
+2022-09-29 17:45:44,Merlan,Pleiades Sector KS-T c3-2,"-75,8125","-100,6875","-292,25",Atlas,"-76,71875","-147,34375","-344,4375",70,N
+2022-09-29 17:48:31,Merlan,Atlas,"-76,71875","-147,34375","-344,4375",HR 1172,"-75,1875","-152,40625","-346,59375","5,7",N
+2022-09-29 19:11:07,Marko Lomic,California Sector LX-T b3-0,"-337,90625","-211,9375","-927,03125",California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875","27,1",N
+2022-09-29 19:30:46,EREBUS5,Slegi QP-Z b58-0,"-1770,90625","-602,0625","-2358,21875",Oochoss LL-D c1,"-1762,46875","-581,84375","-2319,875","44,2",N
+2022-09-29 19:43:34,Yeri-san,Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875",Pleiades Sector VU-O b6-0,"-75,0625","-127,65625","-286,96875","62,8",N
+2022-09-29 20:15:13,Yeri-san,HIP 17168,"-103,75","-128,5625","-329,9375",Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875","17,7",N
+2022-09-29 22:24:21,SZCZYPH,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector PD-S b4-2,"-75,5625","-152,40625","-336,40625",10,N
+2022-09-30 11:29:36,Cannibal cookie,HIP 17999,"-64,0625","-125,5","-296,71875",Asterope,"-80,15625","-144,09375","-333,375","44,1",
+2022-09-30 15:09:18,LilacLight,Col 69 Sector BL-N c7-4,"497,09375","-273,5","-1337,0625",Orion Dark Region CL-Y d17,"509,4375","-277,34375","-1335,78125",13,N
+2022-09-30 16:43:20,LilacLight,Oochost XM-L c24-1,"-262,4375","-149,3125","-1293,59375",Oochost LT-F d12-12,"-265,875","-157,8125","-1292,375","9,2",Y
+2022-09-30 17:01:08,Acred,Pleiades Sector KS-S b4-3,"-62,90625","-91,96875","-339,21875",HIP 22460,"-41,3125","-58,96875","-354,78125","42,4",Y
+2022-09-30 17:19:51,LilacLight,Oochoss SM-B c1-0,"-1712,71875","-597,625","-2268,34375",Oochoss VX-Z c1-0,"-1691,84375","-576,5625","-2225,3125","52,3",N
+2022-09-30 17:47:31,LilacLight,Oochoss PL-P b9-0,"-1642,3125","-563,53125","-2143,75",Oochoss VX-Z c1-0,"-1691,84375","-576,5625","-2225,3125","96,3",N
+2022-09-30 17:55:34,Grimscrub,Oochost AT-J c25-0,"-266,5","-155,90625","-1242,46875",Oochost LT-F d12-12,"-265,875","-157,8125","-1292,375","49,9",N
+2022-09-30 19:11:10,Will keaton,Witch Head Sector UU-O b6-0,"336,4375","-382,40625","-679,625",Siniang,"354,40625","-386,625","-709,21875","34,9",N
+2022-09-30 20:05:12,Helmut Grokenberger,Oochost BT-J c25-0,"-256,5625","-151,9375","-1257,9375",Oochost LT-F d12-12,"-265,875","-157,8125","-1292,375","36,2",N
+2022-09-30 20:14:32,Grimscrub,Oochost XM-L c24-1,"-262,4375","-149,3125","-1293,59375",Oochost LT-F d12-12,"-265,875","-157,8125","-1292,375","9,2",Y
+2022-09-30 20:26:05,Molan Ryke,Col 69 Sector XP-O d6-28,"428,90625","-250,71875","-1124,59375",Col 69 Sector QB-G b26-0,"418,59375","-244,09375","-1103,125","24,7",N
+2022-09-30 21:01:42,Malcotch,Pleiades Sector OS-U c2-6,"-104,3125","-239,25","-336,5625",Pleiades Sector OS-U c2-7,"-68,71875","-229,625","-325,6875","38,4",N
+2022-09-30 21:19:00,Gray Z7Z,Pleiades Sector XF-N b7-1,"-77,09375",-121,"-278,65625",Pleiades Sector PD-S b4-2,"-75,5625","-152,40625","-336,40625","65,8",N
+2022-09-30 23:09:45,Ashurson,Oochost YV-T b50-0,"-261,6875","-143,09375","-1262,84375",Oochost XM-L c24-1,"-262,4375","-149,3125","-1293,59375","31,4",N
+2022-09-30 23:32:23,Hans Acker,Oochoss XI-Y c2-1,"-1700,15625","-544,53125","-2222,25",Oochoss VX-Z c1-0,"-1691,84375","-576,5625","-2225,3125","33,2",N
+2022-09-30 23:54:15,Novaseta,Witch Head Sector DL-Y d13,"389,4375","-400,8125","-732,46875",Witch Head Sector RY-R b4-0,"365,0625","-413,8125","-711,1875","34,9",
+2022-09-30 23:55:50,Novaseta,Witch Head Sector DL-Y d13,"389,4375","-400,8125","-732,46875",Witch Head Sector RY-R b4-0,"365,0625","-413,8125","-711,1875","34,9",
+2022-10-01 01:03:59,Alfredo Cavatelli,Col 69 Sector XV-C c13-10,"434,0625","-283,3125","-1094,3125",Col 69 Sector QB-G b26-0,"418,59375","-244,09375","-1103,125","43,1",N
+2022-10-01 03:07:44,pokegustavo,Col 69 Sector QB-G b26-0,"418,59375","-244,09375","-1103,125",Col 69 Sector LC-V e2-7,"419,625","-242,34375","-1099,03125","4,6",Y
+2022-10-01 03:09:35,Erion Emiroi,Running Man Sector GW-W d1-7,"645,46875","-400,46875","-1034,65625",Col 69 Sector LC-V e2-7,"419,625","-242,34375","-1099,03125","283,1",N
+2022-10-01 03:13:35,Eeka,Delphi,"-63,59375","-147,40625","-319,09375",Pleiades Sector IR-W d1-38,"-35,25","-171,8125","-324,4375","37,8",N
+2022-10-01 03:19:04,pokegustavo,Col 69 Sector LC-V e2-7,"419,625","-242,34375","-1099,03125",Col 69 Sector QB-G b26-0,"418,59375","-244,09375","-1103,125","4,6",N
+2022-10-01 03:22:39,Chase Hutchins,Oochost UP-V b49-0,"-261,875","-141,65625","-1280,53125",Oochost XM-L c24-1,"-262,4375","-149,3125","-1293,59375","15,2",N
+2022-10-01 05:03:49,quinnrz,Oochoss VX-Z c1-0,"-1691,84375","-576,5625","-2225,3125",Oochoss XY-Z d1,"-1698,5","-577,21875","-2227,9375","7,2",Y
+2022-10-01 05:19:18,quinnrz,Oochoss XY-Z d1,"-1698,5","-577,21875","-2227,9375",Oochoss ZD-Y c2-0,"-1701,28125","-581,59375","-2223,75","6,7",N
+2022-10-01 06:59:56,ASFalcon13,Oochost VP-V b49-0,"-241,0625","-140,0625","-1280,34375",Oochost XM-L c24-1,"-262,4375","-149,3125","-1293,59375","26,8",N
+2022-10-01 07:38:53,ASFalcon13,Oochost RE-X b48-0,"-279,375","-164,96875","-1304,875",Oochost LT-F d12-12,"-265,875","-157,8125","-1292,375","19,7",N
+2022-10-01 07:49:51,Ashurson,California Sector ZJ-Z b0,"-304,96875","-204,125","-988,8125",California Sector QD-S b4-0,"-320,0625","-223,34375","-921,625","71,5",N
+2022-10-01 09:18:03,Seven,Col 69 Sector XP-O d6-23,"423,28125","-246,5625","-1099,0625",Col 69 Sector QB-G b26-0,"418,59375","-244,09375","-1103,125","6,7",N
+2022-10-01 09:46:13,Seven,Col 69 Sector XV-C c13-5,"441,53125","-282,875","-1074,15625",Col 69 Sector LC-V e2-7,"419,625","-242,34375","-1099,03125","52,4",N
+2022-10-01 11:19:20,DevilOnTheWall,Musca Dark Region PJ-P b6-1,"432,625","2,53125","288,6875",Musca Dark Region FT-E a13-0,"426,96875","6,5625","287,3125","7,1",N
+2022-10-01 11:26:32,BIA RoS,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Asterope,"-80,15625","-144,09375","-333,375","4,4",N
+2022-10-01 11:44:48,GCSpitfire,Sterope II,"-81,65625","-147,28125","-340,84375",Celaeno,"-81,09375","-148,3125","-337,09375","3,9",N
+2022-10-01 14:12:41,MIBE7070,Aries Dark Region UZ-O b6-1,"-75,1875","-140,84375","-224,53125",Pleiades Sector VU-O b6-3,"-71,78125","-141,375","-294,53125","70,1",N
+2022-10-01 14:33:47,Poperrap,Taurus Dark Region YU-O b6-3,"1,84375","-72,90625","-399,46875",Synuefe HX-B c16-5,"-0,46875","-53,625","-345,03125","57,8",N
+2022-10-01 14:42:46,MIBE7070,Oochost UP-V b49-0,"-261,875","-141,65625","-1280,53125",Oochost XM-L c24-1,"-262,4375","-149,3125","-1293,59375","15,2",N
+2022-10-01 15:07:34,Kongounaut,Col 285 Sector DP-Y b1-3,"-36,21875","-59,6875","-311,53125",Synuefe KD-A c17-10,"-32,375","-58,1875","-332,6875","21,6",Y
+2022-10-01 15:11:49,Kongounaut,Synuefe KD-A c17-10,"-32,375","-58,1875","-332,6875",HIP 22460,"-41,3125","-58,96875","-354,78125","23,8",Y
+2022-10-01 15:44:57,BIA RoS,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","5,2",Y
+2022-10-01 15:50:37,Seven,Col 69 Sector OG-G b26-0,"416,3125","-214,5625","-1098,28125",Col 69 Sector QB-G b26-0,"418,59375","-244,09375","-1103,125",30,N
+2022-10-01 15:51:48,Mass 1nhibited,California Sector HW-W c1-7,"-331,09375","-231,71875","-929,78125",California Sector HW-W c1-4,"-330,71875","-233,59375","-909,1875","20,7",N
+2022-10-01 16:17:38,Kongounaut,Synuefe KD-A c17-9,"-30,6875","-56,90625","-329,96875",Synuefe GX-B c16-6,"-37,25","-55,125","-347,15625","18,5",Y
+2022-10-01 16:24:01,Kongounaut,Synuefe GX-B c16-6,"-37,25","-55,125","-347,15625",Synuefe CT-O d7-73,"-53,5625","-43,9375","-347,71875","19,8",Y
+2022-10-01 16:34:08,CJMVAUCLUSE,Oochoss EK-W c3-0,"-1663,0625","-584,65625","-2181,34375",Oochoss VX-Z c1-0,"-1691,84375","-576,5625","-2225,3125","53,2",N
+2022-10-01 16:35:56,ENERGYMR,Col 69 Sector XV-C c13-2,"430,84375","-274,375","-1097,4375",Col 69 Sector QB-G b26-0,"418,59375","-244,09375","-1103,125","33,2",N
+2022-10-01 16:52:10,Marko Lomic,California Sector HW-W c1-2,"-324,78125","-227,8125","-931,03125",California Sector HR-W d1-25,"-318,9375","-223,40625","-904,96875","27,1",N
+2022-10-01 17:05:34,Marko Lomic,HIP 18390,"-299,0625","-229,25","-876,125",California Sector KH-V c2-11,"-303,53125","-224,34375","-897,71875","22,6",N
+2022-10-01 19:06:25,Cordon Bleu,HD 245680,"427,84375","-295,90625","-1095,25",Col 69 Sector LC-V e2-7,"419,625","-242,34375","-1099,03125","54,3",N
+2022-10-01 19:38:01,quinnrz,Oochoss XY-Z d2,"-1707,84375","-567,59375","-2219,4375",Oochoss ZD-Y c2-0,"-1701,28125","-581,59375","-2223,75","16,1",N
+2022-10-01 21:11:08,Yeri-san,Pleiades Sector IH-V c2-0,"-104,3125","-140,4375","-344,78125",Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875","4,4",N
+2022-10-02 00:30:11,Gray Z7Z,Pleiades Sector VU-O b6-3,"-71,78125","-141,375","-294,53125",Delphi,"-63,59375","-147,40625","-319,09375","26,6",N
+2022-10-02 01:54:35,HENRY KISSINGER,Hyades Sector HM-B a1-0,"-34,125","-34,34375","-273,1875",Synuefe OI-Z a68-0,"-43,21875","-33,0625","-332,8125","60,3",N
+2022-10-02 01:58:36,HENRY KISSINGER,Synuefe OI-Z a68-0,"-43,21875","-33,0625","-332,8125",Synuefe JC-B a68-1,"-45,21875","-34,71875","-338,78125","6,5",Y
+2022-10-02 03:08:06,LilacLight,Oochoss EK-W c3-0,"-1663,0625","-584,65625","-2181,34375",Oochoss PL-P b9-0,"-1642,3125","-563,53125","-2143,75","47,9",N
+2022-10-02 03:19:46,LilacLight,Oochoss OL-P b9-0,"-1659,84375","-564,46875","-2141,375",Oochoss PL-P b9-0,"-1642,3125","-563,53125","-2143,75","17,7",N
+2022-10-02 09:31:26,caRGOrunner1969,HIP 17704,"-72,03125","-133,90625","-311,09375",HR 1183,"-72,875","-145,09375","-336,96875","28,2",
+2022-10-02 09:43:32,padremo,Oochost LT-F d12-2,"-245,6875","-121,96875","-1233,25",Oochost XM-L c24-1,"-262,4375","-149,3125","-1293,59375","68,3",N
+2022-10-02 10:48:12,msTheo,Oochost UP-V b49-0,"-261,875","-141,65625","-1280,53125",Oochost XM-L c24-1,"-262,4375","-149,3125","-1293,59375","15,2",N
+2022-10-02 10:53:54,caRGOrunner1969,Delphi,"-63,59375","-147,40625","-319,09375",HR 1183,"-72,875","-145,09375","-336,96875","20,3",
+2022-10-02 11:51:19,Kongounaut,HR 1554,"-32,375","-57,53125","-340,65625",HR 1477,"-30,625","-81,25","-335,59375","24,3",Y
+2022-10-02 12:28:36,Louis Calvert,Pleiades Sector HR-W d1-79,"-80,625","-146,65625","-343,25",Maia,"-81,78125","-149,4375","-343,375",3,N
+2022-10-02 12:35:22,Kira Nakatani,Pleiades Sector KS-T c3-3,"-77,65625","-97,84375","-290,8125",Pleiades Sector TZ-O b6-2,"-78,40625","-109,09375","-294,78125",12,N
+2022-10-02 12:41:23,Kira Nakatani,Pleiades Sector MN-T c3-12,"-70,65625","-119,75","-302,8125",HIP 17704,"-72,03125","-133,90625","-311,09375","16,5",N
+2022-10-02 12:52:11,Louis Calvert,Maia,"-81,78125","-149,4375","-343,375",Merope,"-78,59375","-149,625","-340,53125","4,3",N
+2022-10-02 13:25:37,LilacLight,Oochoss VN-P c7-0,"-1542,0625","-544,84375",-2024,Oochoss YY-N c8-0,"-1502,1875","-504,09375","-1982,1875","70,7",N
+2022-10-02 15:34:39,LilacLight,Oochoss GA-M c9-0,"-1413,625","-505,71875","-1920,53125",Oochoss OC-T d4-3,"-1440,03125","-499,9375","-1905,125","31,1",N
+2022-10-02 16:32:34,Last winter,Pleiades Sector DL-Y d65,"-83,28125","-151,40625","-347,1875",Asterope,"-80,15625","-144,09375","-333,375","15,9",N
+2022-10-02 17:10:46,quinnrz,Oochoss TI-R d5-3,"-1411,59375",-487,"-1829,03125",Oochoss QX-G c12-0,"-1404,84375","-492,375","-1823,4375","10,3",N
+2022-10-02 17:21:12,quinnrz,Oochoss QX-G c12-0,"-1404,84375","-492,375","-1823,4375",Oochoss TI-R d5-3,"-1411,59375",-487,"-1829,03125","10,3",N
+2022-10-02 17:33:08,quinnrz,Oochoss QX-G c12-0,"-1404,84375","-492,375","-1823,4375",Oochoss TI-R d5-3,"-1411,59375",-487,"-1829,03125","10,3",N
+2022-10-02 17:51:31,pokegustavo,Oochost DC-S b51-0,"-240,71875","-140,71875","-1242,28125",Oochost UP-V b49-0,"-261,875","-141,65625","-1280,53125","43,7",N
+2022-10-02 17:57:15,4lreadyInUs3,HR 1183,"-72,875","-145,09375","-336,96875",Asterope,"-80,15625","-144,09375","-333,375","8,2",N
+2022-10-02 17:58:05,quinnrz,Oochoss TI-R d5-2,"-1418,5","-490,9375","-1838,59375",Oochoss QX-G c12-0,"-1404,84375","-492,375","-1823,4375","20,5",N
+2022-10-02 19:04:57,quinnrz,Oochoss VD-F c13-0,"-1381,75","-499,1875","-1783,75",Oochoss XO-P d6-4,"-1370,53125","-483,53125","-1783,03125","19,3",N
+2022-10-02 19:11:35,LilacLight,Oochoss VD-F c13-0,"-1381,75","-499,1875","-1783,75",Oochoss XO-P d6-4,"-1370,53125","-483,53125","-1783,03125","19,3",N
+2022-10-02 19:22:51,quinnrz,Oochoss XO-P d6-3,"-1374,65625","-467,09375","-1768,40625",Oochoss XO-P d6-4,"-1370,53125","-483,53125","-1783,03125","22,4",N
+2022-10-02 19:29:24,quinnrz,Oochoss XO-P d6-3,"-1374,65625","-467,09375","-1768,40625",Oochoss XO-P d6-4,"-1370,53125","-483,53125","-1783,03125","22,4",N
+2022-10-02 19:39:29,LilacLight,Oochoss XO-P d6-0,"-1414,0625","-500,21875","-1770,25",Oochoss XO-P d6-4,"-1370,53125","-483,53125","-1783,03125","48,3",N
+2022-10-02 19:42:40,Gray Z7Z,Pleiades Sector PD-S b4-2,"-75,5625","-152,40625","-336,40625",Delphi,"-63,59375","-147,40625","-319,09375","21,6",N
+2022-10-02 19:47:38,quinnrz,Oochoss VD-F c13-0,"-1381,75","-499,1875","-1783,75",Oochoss XO-P d6-4,"-1370,53125","-483,53125","-1783,03125","19,3",N
+2022-10-02 19:54:58,Helmut Grokenberger,Pleiades Sector AL-X c1-9,"-82,15625","-54,46875","-359,21875",Pleiades Sector LD-R b5-0,"-75,53125","-70,15625","-305,6875","56,2",Y
+2022-10-02 20:23:30,quinnrz,Oochoss VD-F c13-0,"-1381,75","-499,1875","-1783,75",Oochoss XO-P d6-4,"-1370,53125","-483,53125","-1783,03125","19,3",N
+2022-10-02 20:24:48,LilacLight,Oochoss VD-F c13-0,"-1381,75","-499,1875","-1783,75",Oochoss XO-P d6-4,"-1370,53125","-483,53125","-1783,03125","19,3",N
+2022-10-02 20:32:31,quinnrz,Oochoss VD-F c13-0,"-1381,75","-499,1875","-1783,75",Oochoss XO-P d6-4,"-1370,53125","-483,53125","-1783,03125","19,3",N
+2022-10-02 20:47:05,Istarias,Pleiades Sector RO-Q b5-0,"-80,46875","-140,21875","-324,15625",Asterope,"-80,15625","-144,09375","-333,375",10,N
+2022-10-02 21:03:45,LilacLight,Oochost IO-P d6-2,"-1329,875","-484,65625","-1735,875",Oochost SN-D c14-0,"-1339,53125","-461,90625","-1742,53125","25,6",N
+2022-10-02 21:04:25,quinnrz,Oochost TN-D c14-0,"-1299,21875","-460,5","-1744,375",Oochost SN-D c14-0,"-1339,53125","-461,90625","-1742,53125","40,4",N
+2022-10-02 21:08:57,LilacLight,Oochost IO-P d6-2,"-1329,875","-484,65625","-1735,875",Oochost SN-D c14-0,"-1339,53125","-461,90625","-1742,53125","25,6",N
+2022-10-02 21:12:58,LilacLight,Oochost IO-P d6-2,"-1329,875","-484,65625","-1735,875",Oochost SN-D c14-0,"-1339,53125","-461,90625","-1742,53125","25,6",N
+2022-10-02 21:16:07,LilacLight,Oochost IO-P d6-2,"-1329,875","-484,65625","-1735,875",Oochost SN-D c14-0,"-1339,53125","-461,90625","-1742,53125","25,6",N
+2022-10-02 21:19:29,LilacLight,Oochost IO-P d6-2,"-1329,875","-484,65625","-1735,875",Oochost SN-D c14-0,"-1339,53125","-461,90625","-1742,53125","25,6",N
+2022-10-02 22:32:45,LilacLight,Oochost IO-P d6-2,"-1329,875","-484,65625","-1735,875",Oochost SN-D c14-0,"-1339,53125","-461,90625","-1742,53125","25,6",N
+2022-10-02 22:42:45,LilacLight,Oochost IO-P d6-2,"-1329,875","-484,65625","-1735,875",Oochost SN-D c14-0,"-1339,53125","-461,90625","-1742,53125","25,6",N
+2022-10-02 22:48:03,LilacLight,Oochost IO-P d6-2,"-1329,875","-484,65625","-1735,875",Oochost SN-D c14-0,"-1339,53125","-461,90625","-1742,53125","25,6",N
+2022-10-03 00:07:45,LilacLight,Oochost YO-B c15-0,"-1344,5","-477,75","-1695,65625",Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375","47,6",N
+2022-10-03 00:10:02,LilacLight,Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375",Oochost GX-T e3-0,"-1298,8125","-454,4375","-1693,65625",12,Y
+2022-10-03 00:14:39,LilacLight,Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375",Oochost GX-T e3-0,"-1298,8125","-454,4375","-1693,65625",12,Y
+2022-10-03 00:22:10,LilacLight,Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375",Oochost GX-T e3-0,"-1298,8125","-454,4375","-1693,65625",12,Y
+2022-10-03 00:39:56,LilacLight,Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375",Oochost GX-T e3-0,"-1298,8125","-454,4375","-1693,65625",12,Y
+2022-10-03 00:46:39,LilacLight,Oochost GX-T e3-0,"-1298,8125","-454,4375","-1693,65625",Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375",12,N
+2022-10-03 02:07:04,quinnrz,Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375",Oochost VI-D c14-0,"-1304,875","-479,78125","-1705,0625","19,8",N
+2022-10-03 02:31:40,quinnrz,Oochost VI-D c14-0,"-1304,875","-479,78125","-1705,0625",Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375","19,8",N
+2022-10-03 11:47:55,LilacLight,Oochost KO-O b53-0,"-248,46875","-144,375","-1204,09375",Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375","9,2",N
+2022-10-03 13:02:35,Thommychen,Pleione,-77,"-146,78125","-344,125",Merope,"-78,59375","-149,625","-340,53125","4,9",N
+2022-10-03 13:22:25,The Notorious B.I.G.,Synuefe VG-A b34-3,"-35,71875","-51,65625","-330,15625",HIP 22460,"-41,3125","-58,96875","-354,78125","26,3",N
+2022-10-03 15:56:21,Louis Calvert,Maia,"-81,78125","-149,4375","-343,375",Merope,"-78,59375","-149,625","-340,53125","4,3",N
+2022-10-03 16:49:09,The Notorious B.I.G.,Pleiades Sector JX-S b4-3,"-26,84375","-69,875","-326,9375",Pleiades Sector AL-W b2-1,"-51,78125","-75,3125","-372,375","52,1",N
+2022-10-03 16:56:49,The Notorious B.I.G.,HIP 21283,"-77,8125","-78,53125","-413,53125",HR 1490,"-49,5625","-73,125","-371,125","51,2",N
+2022-10-03 17:01:38,Louis Calvert,Pleiades Sector RO-Q b5-0,"-80,46875","-140,21875","-324,15625",HIP 17704,"-72,03125","-133,90625","-311,09375","16,8",N
+2022-10-03 19:39:58,Merlan,Pleiades Sector UU-O b6-3,"-88,78125","-135,875","-304,59375",Maia,"-81,78125","-149,4375","-343,375","41,7",N
+2022-10-03 19:52:47,quinnrz,Oochost VY-B c15-0,"-1300,3125","-418,5","-1690,1875",Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375","44,1",N
+2022-10-03 19:56:42,quinnrz,Oochost BA-A c16-0,"-1299,375","-464,53125","-1663,71875",Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375","40,4",N
+2022-10-03 20:38:57,quinnrz,Oochost BA-A c16-0,"-1299,375","-464,53125","-1663,71875",Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375","40,4",N
+2022-10-03 20:44:56,quinnrz,Oochost VI-D c14-0,"-1304,875","-479,78125","-1705,0625",Oochost GX-T e3-0,"-1298,8125","-454,4375","-1693,65625","28,4",N
+2022-10-03 21:02:36,quinnrz,Oochost VI-D c14-0,"-1304,875","-479,78125","-1705,0625",Oochost GX-T e3-0,"-1298,8125","-454,4375","-1693,65625","28,4",N
+2022-10-03 21:11:25,quinnrz,Oochost GX-T e3-0,"-1298,8125","-454,4375","-1693,65625",Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375",12,N
+2022-10-03 21:36:08,quinnrz,Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375",Oochost GX-T e3-0,"-1298,8125","-454,4375","-1693,65625",12,Y
+2022-10-04 00:06:14,TheMightySardine,Wolf 1279,"-51,46875","-117,46875","-250,78125",Pleiades Sector HR-W d1-57,"-86,40625","-125,15625","-301,84375","62,3",N
+2022-10-04 00:08:56,TheMightySardine,Pleiades Sector HR-W d1-57,"-86,40625","-125,15625","-301,84375",Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875",46,N
+2022-10-04 01:40:37,Gray Z7Z,Pleiades Sector IH-V c2-18,"-88,34375","-143,78125","-338,84375",Pleiades Sector HR-W d1-42,"-70,1875","-150,21875","-343,84375","19,9",N
+2022-10-04 05:43:52,Roger Acewell,Oochost YV-T b50-0,"-261,6875","-143,09375","-1262,84375",Oochost HI-Q b52-0,"-241,6875","-135,625",-1213,"54,2",N
+2022-10-04 05:52:51,Roger Acewell,Oochost BT-J c25-0,"-256,5625","-151,9375","-1257,9375",Oochost HI-Q b52-0,"-241,6875","-135,625",-1213,"50,1",N
+2022-10-04 12:29:46,Raghav102,Taurus Dark Region TZ-O b6-1,"-47,625","-59,78125","-398,09375",Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875","36,9",Y
+2022-10-04 13:27:53,TheMightySardine,Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875",Asterope,"-80,15625","-144,09375","-333,375","22,8",N
+2022-10-04 15:55:41,Mass 1nhibited,HIP 18390,"-299,0625","-229,25","-876,125",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375","22,2",N
+2022-10-04 16:13:31,LilacLight,Oochost ZG-S b51-0,"-282,5625","-123,625","-1242,34375",Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375","59,8",N
+2022-10-04 16:19:08,DRifter Macraken,Pleiades Sector HW-W c1-10,"-33,625","-162,75","-354,375",HIP 19284,"-25,40625","-164,875","-365,40625","13,9",
+2022-10-04 16:53:24,LilacLight,Oochost QF-M d8-2,"-1148,21875","-371,375","-1598,5625",Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375","205,8",N
+2022-10-04 17:13:45,Epaphus,Col 285 Sector DP-Y b1-4,"-30,46875","-58,625","-305,90625",HIP 22460,"-41,3125","-58,96875","-354,78125","50,1",N
+2022-10-04 17:54:19,Mass 1nhibited,California Sector LC-V c2-3,"-315,40625","-228,84375","-882,59375",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375","10,6",N
+2022-10-04 18:00:20,Henoch,Oochost LT-F d12-2,"-245,6875","-121,96875","-1233,25",Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375","35,2",N
+2022-10-04 18:07:23,LilacLight,Oochost GX-T e3-0,"-1298,8125","-454,4375","-1693,65625",Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375",12,N
+2022-10-04 18:08:05,Roger Acewell,Oochost KO-O b53-0,"-248,46875","-144,375","-1204,09375",Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375","9,2",N
+2022-10-04 18:12:56,LilacLight,Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375",Oochost GX-T e3-0,"-1298,8125","-454,4375","-1693,65625",12,Y
+2022-10-04 18:20:37,LilacLight,Oochost GX-T e3-0,"-1298,8125","-454,4375","-1693,65625",Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375",12,N
+2022-10-04 18:25:55,LilacLight,Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375",Oochost GX-T e3-0,"-1298,8125","-454,4375","-1693,65625",12,Y
+2022-10-04 18:53:56,Bommy,Oochost TA-L b55-0,"-243,0625","-141,59375","-1162,53125",Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375",42,N
+2022-10-04 19:10:09,4lreadyInUs3,Asterope,"-80,15625","-144,09375","-333,375",HR 1183,"-72,875","-145,09375","-336,96875","8,2",N
+2022-10-04 19:15:49,Istarias,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Merope,"-78,59375","-149,625","-340,53125",9,N
+2022-10-04 19:19:11,LilacLight,Oochost NU-N d7-3,"-1248,25","-462,84375","-1667,5625",Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375",64,N
+2022-10-04 19:21:39,Yeri-san,Asterope,"-80,15625","-144,09375","-333,375",Celaeno,"-81,09375","-148,3125","-337,09375","5,7",N
+2022-10-04 19:26:48,Eeka,Oochost HI-Q b52-0,"-241,6875","-135,625",-1213,Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375","10,4",N
+2022-10-04 19:43:49,quinnrz,Oochost UI-D c14-0,"-1316,9375","-483,4375","-1706,875",Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375","28,2",N
+2022-10-04 19:58:23,quinnrz,Oochost GX-T e3-0,"-1298,8125","-454,4375","-1693,65625",Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375",12,N
+2022-10-04 20:02:54,Yeri-san,Maia,"-81,78125","-149,4375","-343,375",HR 1172,"-75,1875","-152,40625","-346,59375","7,9",N
+2022-10-04 20:05:50,quinnrz,Oochost GX-T e3-0,"-1298,8125","-454,4375","-1693,65625",Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375",12,N
+2022-10-04 20:13:42,Eeka,Oochost HI-Q b52-0,"-241,6875","-135,625",-1213,Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375","10,4",N
+2022-10-04 20:19:35,Eeka,Oochost HI-Q b52-0,"-241,6875","-135,625",-1213,Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375","10,4",N
+2022-10-04 20:41:09,Yeri-san,Asterope,"-80,15625","-144,09375","-333,375",Celaeno,"-81,09375","-148,3125","-337,09375","5,7",N
+2022-10-04 20:44:42,Yeri-san,Celaeno,"-81,09375","-148,3125","-337,09375",Asterope,"-80,15625","-144,09375","-333,375","5,7",N
+2022-10-04 21:27:21,Dogsbody D,Yuanjia,"387,9375","-387,6875","-695,4375",HIP 23759,"359,84375","-385,53125","-718,375","36,3",N
+2022-10-04 21:42:08,Will keaton,Pleiades Sector VU-O b6-3,"-71,78125","-141,375","-294,53125",HIP 17692,"-74,125","-143,96875","-328,78125","34,4",N
+2022-10-04 22:02:29,LilacLight,Oochost GX-T e3-0,"-1298,8125","-454,4375","-1693,65625",Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375",12,N
+2022-10-04 22:14:24,DRifter Macraken,California Sector JH-M b7-0,"-304,625","-273,71875","-854,1875",California Sector WO-R b4-0,"-320,25","-284,96875","-922,1875","70,7",
+2022-10-04 22:26:26,Eeka,Oochost HI-Q b52-0,"-241,6875","-135,625",-1213,Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375","10,4",N
+2022-10-04 22:39:54,Eeka,Oochost HI-Q b52-0,"-241,6875","-135,625",-1213,Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375","10,4",N
+2022-10-04 22:43:37,Eeka,Oochost HI-Q b52-0,"-241,6875","-135,625",-1213,Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375","10,4",N
+2022-10-04 22:46:34,Yeri-san,Hyades Sector AQ-Y d93,"-74,59375","-70,9375","-253,96875",Pleiades Sector RE-P b6-0,"-83,09375","-103,5625","-299,53125","56,7",N
+2022-10-04 22:51:06,Eeka,Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375",Oochost KO-O b53-0,"-248,46875","-144,375","-1204,09375","9,2",Y
+2022-10-05 00:15:58,Gray Z7Z,Pleiades Sector PD-S b4-2,"-75,5625","-152,40625","-336,40625",Merope,"-78,59375","-149,625","-340,53125","5,8",N
+2022-10-05 01:26:08,Gray Z7Z,Pleiades Sector KC-V c2-4,"-88,8125","-148,71875","-321,1875",HR 1185,"-64,65625","-148,9375","-330,40625","25,9",N
+2022-10-05 02:09:38,Gaiwecoor II,HIP 22711,"-35,75","-60,84375","-366,90625",HIP 22460,"-41,3125","-58,96875","-354,78125","13,5",Y
+2022-10-05 02:23:47,pokegustavo,Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375",Oochost KO-O b53-0,"-248,46875","-144,375","-1204,09375","9,2",Y
+2022-10-05 02:42:38,PanPiper,Pleiades Sector EG-X c1-5,"-17,34375","-98,21875","-383,46875",HIP 22460,"-41,3125","-58,96875","-354,78125","54,2",N
+2022-10-05 03:01:54,PanPiper,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector GW-W d1-84,"-58,96875","-99,21875","-336,59375","47,6",Y
+2022-10-05 03:20:25,PanPiper,HIP 21852,"-38,53125","-75,3125","-352,40625",HIP 22460,"-41,3125","-58,96875","-354,78125","16,7",Y
+2022-10-05 04:56:05,Dream Shift,Hyades Sector NS-B b4,"-28,9375","-14,8125","-268,71875",Col 285 Sector WW-A a2-2,"-42,84375","-41,0625","-328,9375","67,1",N
+2022-10-05 04:58:26,Dream Shift,Col 285 Sector WW-A a2-2,"-42,84375","-41,0625","-328,9375",Taurus Dark Region MP-C a14-1,"-59,21875","-44,75","-391,15625","64,4",Y
+2022-10-05 04:59:14,Salayyer,Oochost NU-N d7-3,"-1248,25","-462,84375","-1667,5625",Oochost GX-T e3-0,"-1298,8125","-454,4375","-1693,65625","57,5",
+2022-10-05 08:13:39,HugoHabicht,Oochost HI-Q b52-0,"-241,6875","-135,625",-1213,Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375","10,4",N
+2022-10-05 08:25:27,HugoHabicht,California Sector RD-S b4-0,"-302,78125","-222,1875","-920,0625",HIP 18390,"-299,0625","-229,25","-876,125","44,7",N
+2022-10-05 13:07:56,Gregor Adama,Delphi,"-63,59375","-147,40625","-319,09375",HIP 17125,"-95,875","-128,75","-316,5625","37,4",N
+2022-10-05 14:34:37,NotAllHere,Pleiades Sector ER-U b3-4,"-47,375","-83,03125","-359,875",Pleiades Sector FR-U b3-0,"-38,4375",-69,"-359,96875","16,6",
+2022-10-05 15:20:37,Mass 1nhibited,HIP 18390,"-299,0625","-229,25","-876,125",California Sector LC-V c2-3,"-315,40625","-228,84375","-882,59375","17,6",N
+2022-10-05 18:45:46,DRifter Macraken,Pleione,-77,"-146,78125","-344,125",Pleiades Sector GH-V c2-9,"-149,1875","-132,65625","-339,78125","73,7",
+2022-10-05 19:12:29,GrayC,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Pleiades Sector GW-W c1-15,"-76,9375","-153,71875","-346,625","16,1",N
+2022-10-05 19:25:33,msTheo,Oochost KO-O b53-0,"-248,46875","-144,375","-1204,09375",Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375","9,2",N
+2022-10-05 19:29:15,Grimscrub,Col 285 Sector PC-T b4-2,"-82,46875","-82,96875","-258,59375",Pleiades Sector TZ-O b6-3,"-74,375","-115,71875","-294,53125","49,3",N
+2022-10-05 19:34:01,Grimscrub,Pleiades Sector TZ-O b6-3,"-74,375","-115,71875","-294,53125",HIP 17692,"-74,125","-143,96875","-328,78125","44,4",N
+2022-10-05 20:20:55,Malcotch,Pleiades Sector OI-T c3-7,"-69,4375","-180,4375","-287,625",Pleiades Sector IR-W d1-61,"-50,09375","-180,84375","-291,46875","19,7",N
+2022-10-05 20:35:20,INOSAK,Oochost LT-F d12-2,"-245,6875","-121,96875","-1233,25",Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375","35,2",N
+2022-10-05 20:40:54,Thommychen,Taygeta,"-88,5","-159,6875","-366,28125",HIP 17225,"-86,15625","-165,59375","-356,8125","11,4",N
+2022-10-05 21:12:28,Thommychen,Taygeta,"-88,5","-159,6875","-366,28125",Asterope,"-80,15625","-144,09375","-333,375","37,4",N
+2022-10-05 21:36:15,GrayC,Pleiades Sector HR-W d1-74,"-87,40625","-150,90625","-344,96875",HIP 16753,"-88,3125","-172,9375","-348,84375","22,4",N
+2022-10-05 22:17:34,Eeka,Oochost HI-Q b52-0,"-241,6875","-135,625",-1213,Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375","10,4",N
+2022-10-05 22:21:02,Eeka,Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375",Oochost HI-Q b52-0,"-241,6875","-135,625",-1213,"10,4",Y
+2022-10-05 22:37:12,pokegustavo,Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375",Oochost JT-O b53-0,"-240,1875","-124,21875","-1202,1875","17,2",Y
+2022-10-05 23:01:03,Eeka,Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375",Oochost HI-Q b52-0,"-241,6875","-135,625",-1213,"10,4",Y
+2022-10-05 23:04:07,pokegustavo,Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375",Oochost KO-O b53-0,"-248,46875","-144,375","-1204,09375","9,2",Y
+2022-10-05 23:06:21,pokegustavo,Oochost KO-O b53-0,"-248,46875","-144,375","-1204,09375",Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375","9,2",N
+2022-10-05 23:09:10,Istarias,Merope,"-78,59375","-149,625","-340,53125",Celaeno,"-81,09375","-148,3125","-337,09375","4,4",N
+2022-10-05 23:09:45,pokegustavo,Oochost KO-O b53-0,"-248,46875","-144,375","-1204,09375",Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375","9,2",N
+2022-10-05 23:19:03,pokegustavo,Oochost KO-O b53-0,"-248,46875","-144,375","-1204,09375",Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375","9,2",N
+2022-10-05 23:33:58,pokegustavo,Oochost KO-O b53-0,"-248,46875","-144,375","-1204,09375",Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375","9,2",N
+2022-10-06 11:31:36,Foperider,Oochost NJ-O b53-0,"-229,46875","-152,59375","-1190,71875",Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375","20,6",N
+2022-10-06 12:36:32,HunabKU,Oochost NU-N d7-3,"-1248,25","-462,84375","-1667,5625",Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375",64,N
+2022-10-06 12:51:43,HunabKU,Oochost NU-N d7-3,"-1248,25","-462,84375","-1667,5625",Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375",64,N
+2022-10-06 13:57:55,Mass 1nhibited,HIP 18077,"-303,84375","-236,125","-860,59375",HIP 18390,"-299,0625","-229,25","-876,125","17,6",N
+2022-10-06 14:39:06,Mass 1nhibited,California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375",California Sector UJ-Q b5-0,"-319,6875","-222,3125","-899,9375",11,N
+2022-10-06 15:05:07,Firedrax,Taurus Dark Region KC-V b2-0,"-121,625","-162,96875","-481,34375",Pleiades Sector ZE-Z b1,"-84,40625","-162,90625","-424,875","67,6",N
+2022-10-06 16:48:49,Merlan,Delphi,"-63,59375","-147,40625","-319,09375",HR 1183,"-72,875","-145,09375","-336,96875","20,3",N
+2022-10-06 17:02:32,Merlan,Pleiades Sector JH-V c2-3,"-59,21875","-109,75","-316,28125",Pleiades Sector SE-P b6-3,"-59,40625","-97,125",-296,"23,9",N
+2022-10-06 19:34:04,Dragoniel,HIP 17403,"-93,6875","-158,96875","-367,625",Pleiades Sector UU-O b6-1,"-86,15625","-138,21875","-302,8125","68,5",N
+2022-10-06 20:15:25,Eeka,Oochost VI-D c14-0,"-1304,875","-479,78125","-1705,0625",Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375","19,8",N
+2022-10-06 20:39:05,Malcotch,Pleiades Sector GW-W c1-15,"-76,9375","-153,71875","-346,625",HIP 17862,"-81,4375","-151,90625","-359,59375","13,8",N
+2022-10-06 20:50:35,Eeka,Oochost VI-D c14-0,"-1304,875","-479,78125","-1705,0625",Oochost XT-B c15-0,"-1300,96875","-460,375","-1703,84375","19,8",N
+2022-10-06 20:58:24,Deflora,Pleiades Sector EL-Y d36,"-49,625","-161,1875","-398,46875",Pleiades Sector QD-S b4-2,"-49,03125","-155,03125","-340,1875","58,6",N
+2022-10-06 22:41:44,LtNagae,Celaeno,"-81,09375","-148,3125","-337,09375",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","4,6",N
+2022-10-06 23:11:11,LilacLight,Oochost NU-N d7-4,"-1238,53125","-466,28125","-1679,78125",Oochost NU-N d7-3,"-1248,25","-462,84375","-1667,5625",16,N
+2022-10-07 00:40:21,Thyrannis,Oochost MO-O b53-0,"-217,53125","-139,125","-1189,78125",Oochost LO-O b53-0,"-239,84375","-141,25","-1204,4375","26,8",N
+2022-10-07 00:51:56,LilacLight,Oochost NU-N d7-4,"-1238,53125","-466,28125","-1679,78125",Oochost NU-N d7-3,"-1248,25","-462,84375","-1667,5625",16,N
+2022-10-07 01:07:20,LilacLight,Oochost RA-M d8-1,"-1216,5","-459,375","-1608,875",Oochost HG-Y c16-0,"-1222,21875","-460,8125","-1620,25","12,8",N
+2022-10-07 02:37:31,LilacLight,Oochost HG-Y c16-0,"-1222,21875","-460,8125","-1620,25",Oochost RA-M d8-1,"-1216,5","-459,375","-1608,875","12,8",Y
+2022-10-07 02:41:10,LilacLight,Oochost RA-M d8-1,"-1216,5","-459,375","-1608,875",Oochost HG-Y c16-0,"-1222,21875","-460,8125","-1620,25","12,8",N
+2022-10-07 03:10:26,LilacLight,Oochost RA-M d8-3,"-1188,65625","-434,65625","-1570,25",Oochost RA-M d8-4,"-1186,03125","-442,28125","-1568,9375","8,2",Y
+2022-10-07 03:54:36,LilacLight,Oochost KR-W c17-0,"-1181,09375","-421,96875","-1583,03125",Oochost RA-M d8-3,"-1188,65625","-434,65625","-1570,25","19,5",N
+2022-10-07 05:11:55,LilacLight,Oochost OX-U c18-0,"-1182,78125","-423,15625","-1539,09375",Oochost UL-K d9-0,-1173,"-422,40625","-1540,9375",10,N
+2022-10-07 11:43:24,Mass 1nhibited,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector NS-T b3-0,"-344,5625","-243,65625","-940,84375","45,7",N
+2022-10-07 12:17:23,Mass 1nhibited,California Sector HW-W c1-4,"-330,71875","-233,59375","-909,1875",California Sector HR-W d1-28,"-329,53125","-218,5","-893,71875","21,6",N
+2022-10-07 12:18:22,HunabKU,Oochost RA-M d8-3,"-1188,65625","-434,65625","-1570,25",Oochost UL-K d9-0,-1173,"-422,40625","-1540,9375","35,4",N
+2022-10-07 12:34:59,Serraphix,Oochost UL-K d9-1,"-1179,375","-411,5","-1540,5625",Oochost UL-K d9-0,-1173,"-422,40625","-1540,9375","12,6",N
+2022-10-07 15:13:34,HunabKU,Oochost OX-U c18-0,"-1182,78125","-423,15625","-1539,09375",Oochost UL-K d9-0,-1173,"-422,40625","-1540,9375",10,N
+2022-10-07 15:57:21,HunabKU,Oochost RA-M d8-3,"-1188,65625","-434,65625","-1570,25",Oochost UL-K d9-0,-1173,"-422,40625","-1540,9375","35,4",N
+2022-10-07 19:30:27,Helmut Grokenberger,Oochost VK-M b54-0,"-202,28125","-180,03125","-1179,8125",Oochost MQ-E c28-1,"-223,5","-144,78125","-1144,5625","54,2",N
+2022-10-07 20:45:50,Istarias,Celaeno,"-81,09375","-148,3125","-337,09375",Merope,"-78,59375","-149,625","-340,53125","4,4",N
+2022-10-07 21:21:18,Istarias,Merope,"-78,59375","-149,625","-340,53125",Celaeno,"-81,09375","-148,3125","-337,09375","4,4",N
+2022-10-07 21:43:16,Malcotch,Pleiades Sector KC-U b3-1,"-62,40625","-133,0625","-360,875",Pleiades Sector JC-U b3-3,"-70,6875","-138,75","-358,84375","10,2",N
+2022-10-07 21:47:14,Nathan Kerbonaut,Col 285 Sector KP-V a4-0,"-22,34375","-43,4375","-304,34375",Taurus Dark Region XB-Z a15-0,"-32,5625","-43,71875","-374,09375","70,5",N
+2022-10-07 22:38:10,Istarias,Merope,"-78,59375","-149,625","-340,53125",Celaeno,"-81,09375","-148,3125","-337,09375","4,4",N
+2022-10-07 23:58:41,LilacLight,Outorst ZE-H c10-2,"-2102,1875","98,3125","-1893,75",Outorst YJ-R d4-10,"-2083,84375","90,6875","-1911,3125","26,5",N
+2022-10-08 00:41:08,Helmut Grokenberger,Outotch AJ-Y b15-0,"1911,75","216,28125","-1988,375",Outotch UI-T d3-5,"1887,15625","211,625","-1977,21875","27,4",N
+2022-10-08 01:20:39,LilacLight,Oochost PS-U c18-0,"-1186,1875","-430,59375","-1536,5",Oochost UL-K d9-0,-1173,"-422,40625","-1540,9375","16,1",N
+2022-10-08 05:03:06,Sammi Reid,Oochost GT-F b58-0,"-210,21875","-129,25","-1100,15625",Oochost CN-H b57-0,"-222,71875","-134,8125","-1106,03125","14,9",N
+2022-10-08 05:37:22,Reyedog,Musca Dark Region HM-V c2-29,"418,46875","-4,5625","270,65625",Musca Dark Region PJ-P b6-1,"432,625","2,53125","288,6875",24,N
+2022-10-08 05:40:28,Sammi Reid,Outotch MB-N c7-1,"1935,03125","220,03125","-2022,34375",Outotch UI-T d3-5,"1887,15625","211,625","-1977,21875","66,3",N
+2022-10-08 06:13:50,Sageking098,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","4,4",N
+2022-10-08 06:29:37,Sageking098,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Asterope,"-80,15625","-144,09375","-333,375","4,4",N
+2022-10-08 09:32:39,Helmut Grokenberger,Outotch ZI-Y b15-0,"1882,1875","215,46875","-1991,46875",Outotch UI-T d3-5,"1887,15625","211,625","-1977,21875","15,6",N
+2022-10-08 10:35:31,Nora Faye,Oochost MQ-E c28-0,"-224,09375","-139,09375","-1143,09375",Oochost GT-F b58-0,"-210,21875","-129,25","-1100,15625","46,2",N
+2022-10-08 11:49:36,Chundaman,Oochost PX-U c18-0,"-1136,8125","-407,4375","-1523,40625",Oochost UL-K d9-0,-1173,"-422,40625","-1540,9375","42,9",N
+2022-10-08 12:06:33,Space Mickk,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector QO-Q b5-2,"-90,5625","-136,46875","-316,59375","21,2",N
+2022-10-08 13:04:29,Shaye Blackwood,Col 285 Sector FU-V a4-0,"-45,5","-31,78125","-298,03125",Synuefe GX-B c16-6,"-37,25","-55,125","-347,15625",55,N
+2022-10-08 13:07:44,Shaye Blackwood,Synuefe GX-B c16-6,"-37,25","-55,125","-347,15625",HIP 22460,"-41,3125","-58,96875","-354,78125","9,5",Y
+2022-10-08 14:32:52,Istarias,Merope,"-78,59375","-149,625","-340,53125",Celaeno,"-81,09375","-148,3125","-337,09375","4,4",N
+2022-10-08 19:56:25,Mallchad,Pleiades Sector HR-W d1-57,"-86,40625","-125,15625","-301,84375",Pleiades Sector TZ-O b6-0,"-80,40625","-124,125","-304,125","6,5",N
+2022-10-08 20:59:38,Eeka,Pleiades Sector DG-X c1-11,"-49,25","-70,4375","-360,8125",HIP 22460,"-41,3125","-58,96875","-354,78125","15,2",Y
+2022-10-08 23:49:39,Mallchad,Pleiades Sector RO-Q b5-2,"-67,03125","-136,5625","-308,09375",Pleiades Sector WU-O b6-1,"-59,21875","-127,5625","-304,84375","12,4",N
+2022-10-09 00:44:25,Mallchad,Delphi,"-63,59375","-147,40625","-319,09375",HR 1185,"-64,65625","-148,9375","-330,40625","11,5",N
+2022-10-09 01:14:47,Mallchad,HR 1185,"-64,65625","-148,9375","-330,40625",Delphi,"-63,59375","-147,40625","-319,09375","11,5",N
+2022-10-09 02:20:20,Alfredo Cavatelli,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector OI-S b4-0,"-63,1875","-135,75","-325,21875","20,6",N
+2022-10-09 03:16:03,Sammi Reid,Outotch YO-R d4-3,"1895,6875","204,59375","-1918,1875",Outotch SN-J c9-0,"1856,21875","220,1875","-1941,5625","48,4",N
+2022-10-09 06:49:48,Mass 1nhibited,HIP 18077,"-303,84375","-236,125","-860,59375",California Sector UJ-Q b5-0,"-319,6875","-222,3125","-899,9375","44,6",N
+2022-10-09 07:20:47,Mass 1nhibited,HIP 18077,"-303,84375","-236,125","-860,59375",California Sector UJ-Q b5-0,"-319,6875","-222,3125","-899,9375","44,6",N
+2022-10-09 13:12:48,Elorc,Pleiades Sector LC-V c2-8,"-57,5","-164,09375","-312,53125",Pleiades Sector HR-W d1-36,"-67,65625","-150,71875","-333,3125","26,7",N
+2022-10-09 14:15:56,Firedrax,Pleiades Sector PT-Q b5-2,"-74,90625","-119,1875","-313,96875",Pleiades Sector VK-N b7-3,"-75,40625","-104,59375",-280,37,N
+2022-10-09 14:16:48,Seph Iridan,Pleiades Sector MX-U d2-69,"-53,90625","-157,46875","-251,375",Pleiades Sector OI-T c3-7,"-69,4375","-180,4375","-287,625","45,6",N
+2022-10-09 14:49:00,Seph Iridan,Pleiades Sector IX-S b4-1,"-60,78125","-79,53125","-344,90625",Pleiades Sector IX-S b4-4,"-53,125","-71,6875","-337,8125","13,1",Y
+2022-10-09 15:19:36,Mass 1nhibited,California Sector LC-V c2-4,"-318,34375","-230,75","-888,21875",California Sector ON-T c3-0,"-301,71875","-224,78125","-863,3125","30,5",N
+2022-10-09 17:56:53,Mass 1nhibited,Delphi,"-63,59375","-147,40625","-319,09375",Pleiades Sector GW-W c1-12,"-79,90625","-159,09375","-352,78125","39,2",N
+2022-10-09 19:03:21,Cate Nicholls,Col 285 Sector CV-Y d64,"-57,59375","-39,46875","-308,875",HIP 22241,"-57,1875","-51,90625","-356,15625","48,9",N
+2022-10-09 20:44:01,Cate Nicholls,Taurus Dark Region GW-W d1-32,"-52,59375","-60,375","-411,6875",Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875","50,8",N
+2022-10-09 21:00:22,Seph Iridan,Pleiades Sector KS-T c3-14,"-67,65625","-103,59375","-290,90625",Asterope,"-80,15625","-144,09375","-333,375",60,N
+2022-10-09 21:04:35,Seph Iridan,Asterope,"-80,15625","-144,09375","-333,375",Taygeta,"-88,5","-159,6875","-366,28125","37,4",N
+2022-10-09 21:45:07,LobaDK,Oochost OL-E c28-2,"-219,3125","-145,28125","-1111,53125",Oochost CN-H b57-0,"-222,71875","-134,8125","-1106,03125","12,3",N
+2022-10-10 08:46:46,Foperider,Outotch YU-L b22-0,"1775,40625","204,0625",-1854,Outotch XO-R d4-3,"1800,25","204,4375","-1887,5","41,7",N
+2022-10-10 08:57:34,Mass 1nhibited,California Sector LC-V c2-4,"-318,34375","-230,75","-888,21875",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","28,9",N
+2022-10-10 09:29:31,Foperider,Outotch QI-P b20-0,"1780,0625","196,5625","-1886,34375",Outotch YU-L b22-0,"1775,40625","204,0625",-1854,"33,5",N
+2022-10-10 09:52:31,Mass 1nhibited,California Sector UJ-Q b5-0,"-319,6875","-222,3125","-899,9375",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","14,6",N
+2022-10-10 10:15:21,Mass 1nhibited,Perseus Dark Region RT-R c4-2,"-328,84375","-289,40625","-982,59375",California Sector MH-V b2-0,"-317,15625","-251,96875","-956,28125","47,2",N
+2022-10-10 11:10:06,Mass 1nhibited,California Sector UJ-Q b5-0,"-319,6875","-222,3125","-899,9375",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","14,6",N
+2022-10-10 11:33:48,Poperrap,Outotch AV-L b22-0,"1818,65625","207,28125","-1855,6875",Outotch YU-L b22-0,"1775,40625","204,0625",-1854,"43,4",N
+2022-10-10 11:44:55,Mass 1nhibited,California Sector MH-V b2-0,"-317,15625","-251,96875","-956,28125",California Sector IW-W c1-5,"-303,15625","-246,3125","-912,21875","46,6",N
+2022-10-10 12:40:14,Mass 1nhibited,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector HR-W d1-28,"-329,53125","-218,5","-893,71875","22,1",N
+2022-10-10 13:34:51,Mass 1nhibited,California Sector HR-W d1-28,"-329,53125","-218,5","-893,71875",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","22,1",N
+2022-10-10 18:22:26,Serraphix,Pleiades Sector TE-Y b1-4,"-118,125","-82,96875","-393,875",Pleiades Sector BQ-Y d59,"-99,8125","-71,90625","-367,5625","33,9",N
+2022-10-10 18:47:21,Cate Nicholls,Oochost KZ-D b59-0,"-216,5","-134,5","-1066,0625",Oochost EY-F b58-0,"-221,125","-121,34375","-1104,8125","41,2",N
+2022-10-10 19:31:19,Kongounaut,Pleiades Sector EL-Y d65,"-33,28125","-148,6875","-361,1875",HIP 19847,"-24,84375","-153,46875","-377,46875",19,N
+2022-10-10 20:21:28,NeDelebreton,Pleiades Sector VK-N b7-4,"-72,34375","-90,8125","-272,96875",Pleiades Sector HR-W d1-59,"-76,03125","-128,3125","-326,25","65,3",N
+2022-10-10 21:30:59,Kongounaut,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",Y
+2022-10-10 21:57:50,Kongounaut,Pleiades Sector GW-W d1-61,"-59,1875","-103,90625","-340,15625",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","46,6",N
+2022-10-10 22:27:14,Istarias,Pleiades Sector IR-W d1-51,"-52,0625","-112,84375","-267,875",Pleiades Sector RO-Q b5-0,"-80,46875","-140,21875","-324,15625","68,7",N
+2022-10-10 22:31:07,Istarias,Pleiades Sector RO-Q b5-0,"-80,46875","-140,21875","-324,15625",Asterope,"-80,15625","-144,09375","-333,375",10,N
+2022-10-11 00:02:39,Sammi Reid,Flame Sector XJ-A d7,"364,0625","-229,8125",-911,Flame Sector UZ-X b1-0,"368,78125","-216,875","-907,46875","14,2",N
+2022-10-11 03:11:37,HENRY KISSINGER,HR 1183,"-72,875","-145,09375","-336,96875",Pleione,-77,"-146,78125","-344,125","8,4",N
+2022-10-11 09:42:06,Poperrap,Oochost BS-H b57-0,"-199,53125","-119,53125","-1121,6875",Oochost GT-F b58-0,"-210,21875","-129,25","-1100,15625","25,9",N
+2022-10-11 10:30:36,Sammi Reid,Outotch DB-E c12-0,"1760,25","191,125","-1805,8125",Outotch IN-G b25-0,"1735,40625","198,375","-1803,96875","25,9",N
+2022-10-11 14:08:48,Petro UA,Synuefe VG-A b34-3,"-35,71875","-51,65625","-330,15625",HIP 22460,"-41,3125","-58,96875","-354,78125","26,3",N
+2022-10-11 18:16:34,MGFrank70,Pleiades Sector HR-W d1-79,"-80,625","-146,65625","-343,25",HIP 17692,"-74,125","-143,96875","-328,78125","16,1",N
+2022-10-11 18:44:03,Mass 1nhibited,California Sector UJ-Q b5-0,"-319,6875","-222,3125","-899,9375",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","14,6",N
+2022-10-11 19:22:52,Mass 1nhibited,California Sector HR-W d1-28,"-329,53125","-218,5","-893,71875",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375","15,9",N
+2022-10-11 19:24:08,Helmut Grokenberger,Outotch KT-E b26-0,"1698,8125","200,25","-1765,71875",Outotch IN-G b25-0,"1735,40625","198,375","-1803,96875",53,N
+2022-10-12 03:59:21,PanPiper,HIP 18390,"-299,0625","-229,25","-876,125",California Sector XE-Q b5-0,"-303,75","-244,46875","-899,6875","28,4",N
+2022-10-12 04:27:35,PanPiper,California Sector LC-V c2-9,"-322,5625","-239,03125","-892,46875",California Sector DL-Y d16,"-322,1875","-258,3125","-936,125","47,7",N
+2022-10-12 04:56:50,PanPiper,California Sector FB-X c1-7,"-340,15625","-211,65625","-927,9375",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","25,5",N
+2022-10-12 05:58:15,Sammi Reid,M34 Sector YU-Y d3,"-1050,1875","-369,5","-1353,25",M34 Sector VF-V b3-0,"-1040,53125","-384,96875","-1363,0625","20,7",N
+2022-10-12 12:09:49,Mass 1nhibited,California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","45,3",N
+2022-10-12 12:12:15,Mass 1nhibited,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375","24,3",N
+2022-10-12 12:36:50,Arcanic,Pleiades Sector MN-T c3-12,"-70,65625","-119,75","-302,8125",Asterope,"-80,15625","-144,09375","-333,375","40,2",N
+2022-10-12 12:50:49,Arcanic,Celaeno,"-81,09375","-148,3125","-337,09375",Pleione,-77,"-146,78125","-344,125","8,3",N
+2022-10-12 13:57:42,Mass 1nhibited,California Sector EL-Y d22,"-303,40625","-237,75","-913,21875",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375",30,N
+2022-10-12 14:19:35,Arcanic,HIP 17000,"-84,65625","-171,75","-353,125",Asterope,"-80,15625","-144,09375","-333,375","34,3",N
+2022-10-12 14:20:14,Mass 1nhibited,California Sector HR-W d1-28,"-329,53125","-218,5","-893,71875",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","22,1",N
+2022-10-12 18:09:10,Arcanic,Asterope,"-80,15625","-144,09375","-333,375",HIP 14971,"-85,09375","-221,28125","-324,28125","77,9",N
+2022-10-13 01:59:58,Sammi Reid,Synuefai DZ-F b3-0,"-200,875","-123,90625","-1004,03125",Synuefai DZ-F b3-1,"-197,8125","-124,71875",-1000,"5,1",N
+2022-10-13 03:41:57,LilacLight,Flame Sector BQ-Y d21,"349,375","-222,5","-877,28125",Flame Sector CM-U b3-0,"355,84375","-223,625","-881,90625",8,N
+2022-10-13 06:42:08,LilacLight,Flame Sector CM-U b3-0,"355,84375","-223,625","-881,90625",Horsehead Sector GS-S b4-0,"346,1875","-206,6875","-822,78125","62,3",N
+2022-10-13 11:30:30,Mass 1nhibited,California Sector MC-V c2-2,"-300,03125","-244,0625","-865,96875",HIP 18077,"-303,84375","-236,125","-860,59375","10,3",N
+2022-10-13 13:07:22,Mass 1nhibited,California Sector HR-W d1-28,"-329,53125","-218,5","-893,71875",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","22,1",N
+2022-10-13 14:07:08,Mass 1nhibited,California Sector GH-L b8-0,"-279,65625","-199,75","-841,8125",California Sector XU-O b6-0,"-299,46875","-204,875","-880,15625","43,5",N
+2022-10-13 14:11:06,Mass 1nhibited,California Sector XU-O b6-0,"-299,46875","-204,875","-880,15625",California Sector HR-W d1-28,"-329,53125","-218,5","-893,71875","35,7",N
+2022-10-13 15:18:23,Mass 1nhibited,California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","45,3",N
+2022-10-13 15:27:09,SturmWaffel,Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875",Asterope,"-80,15625","-144,09375","-333,375","22,8",N
+2022-10-13 16:17:37,Mass 1nhibited,California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","45,3",N
+2022-10-13 17:32:05,Helmut Grokenberger,Outotch EH-I b24-0,"1737,4375","196,75",-1822,Outotch IN-G b25-0,"1735,40625","198,375","-1803,96875","18,2",N
+2022-10-13 18:39:00,AHappyClownFish,HR 1183,"-72,875","-145,09375","-336,96875",Asterope,"-80,15625","-144,09375","-333,375","8,2",N
+2022-10-13 18:55:25,HydrazineN2H4,Witch Head Sector KC-V c2-4,"360,84375","-396,96875","-704,6875",Witch Head Sector DL-Y d22,"378,21875","-407,65625","-722,90625","27,4",N
+2022-10-13 19:43:01,HydrazineN2H4,California Sector GB-X c1-2,"-286,125","-208,375","-913,21875",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","34,7",N
+2022-10-13 20:29:08,HydrazineN2H4,California Sector GW-W c1-7,"-354,625","-233,6875","-918,4375",California Sector QD-S b4-0,"-320,0625","-223,34375","-921,625","36,2",N
+2022-10-13 20:55:15,allan mandragoran,Arietis Sector DV-Y b0,"-61,90625","-101,46875","-199,90625",Pleiades Sector XF-N b7-2,"-81,6875","-124,03125","-276,71875","82,5",N
+2022-10-13 21:13:34,PanPiper,HIP 17225,"-86,15625","-165,59375","-356,8125",Maia,"-81,78125","-149,4375","-343,375","21,5",N
+2022-10-13 22:53:25,Sammi Reid,Synuefai JL-C b5-1,"-239,59375","-121,75","-957,6875",Synuefai DZ-F b3-1,"-197,8125","-124,71875",-1000,"59,5",N
+2022-10-14 00:45:38,GrayC,HIP 17892,"-70,75","-157,9375","-352,84375",Electra,"-86,0625","-159,9375","-361,65625","17,8",N
+2022-10-14 01:49:31,Mass 1nhibited,California Sector JH-V c2-2,"-328,28125",-202,"-878,25",California Sector FB-X c1-8,"-342,90625","-208,34375","-932,3125","56,4",N
+2022-10-14 04:13:02,allan mandragoran,Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875",Maia,"-81,78125","-149,4375","-343,375","20,9",N
+2022-10-14 09:52:12,Poperrap,Outotch DB-E c12-1,"1753,34375","213,4375","-1816,5",Outotch IN-G b25-0,"1735,40625","198,375","-1803,96875","26,6",N
+2022-10-14 09:55:58,Neutronic1,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector KC-V c2-14,"-85,5","-156,28125","-342,25",16,N
+2022-10-14 10:13:11,Poperrap,Outotch CB-E c12-1,"1712,125","190,96875","-1794,59375",Outotch HN-G b25-0,"1718,53125","203,0625","-1791,3125","14,1",N
+2022-10-14 10:41:48,dart frog,M34 Sector WU-X c1-1,"-991,8125","-344,75","-1363,625",M34 Sector VF-V b3-0,"-1040,53125","-384,96875","-1363,0625","63,2",N
+2022-10-14 11:23:45,Mass 1nhibited,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector HR-W d1-28,"-329,53125","-218,5","-893,71875","22,1",N
+2022-10-14 13:04:33,Neutronic1,Merope,"-78,59375","-149,625","-340,53125",Sterope II,"-81,65625","-147,28125","-340,84375","3,9",N
+2022-10-14 13:31:28,Neutronic1,HIP 15980,"-77,28125","-113,6875","-234,5",Pleiades Sector VU-O b6-3,"-71,78125","-141,375","-294,53125","66,3",N
+2022-10-14 14:32:56,Mass 1nhibited,California Sector LC-V c2-3,"-315,40625","-228,84375","-882,59375",California Sector HR-W d1-25,"-318,9375","-223,40625","-904,96875","23,3",N
+2022-10-14 15:14:40,SturmWaffel,Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875",Asterope,"-80,15625","-144,09375","-333,375","22,8",N
+2022-10-14 16:46:11,Mass 1nhibited,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector LC-V c2-3,"-315,40625","-228,84375","-882,59375","33,5",N
+2022-10-14 17:34:19,ENERGYMR,Delphi,"-63,59375","-147,40625","-319,09375",HIP 17225,"-86,15625","-165,59375","-356,8125","47,6",N
+2022-10-14 19:28:26,Bevien Irnguard,Atlas,"-76,71875","-147,34375","-344,4375",Pleione,-77,"-146,78125","-344,125","0,7",N
+2022-10-14 20:39:18,badloop1,Musca Dark Region CQ-V a3-7,"457,90625","-30,71875","194,15625",Musca Dark Region GM-U b3-5,"444,28125","-18,46875","215,46875","28,1",N
+2022-10-14 20:43:31,badloop1,Musca Dark Region JS-S b4-2,"429,40625","-10,84375","239,1875",Musca Dark Region LD-R b5-2,"416,71875","3,0625","260,8125","28,7",N
+2022-10-14 20:52:34,badloop1,HIP 62154,"411,375","9,09375","261,46875",Coalsack Sector KN-S b4-6,"410,75","35,6875","263,03125","26,6",N
+2022-10-14 21:19:58,Bevien Irnguard,Pleione,-77,"-146,78125","-344,125",Celaeno,"-81,09375","-148,3125","-337,09375","8,3",N
+2022-10-14 21:22:35,msTheo,Synuefai QX-A d1-12,"-195,6875","-122,9375","-964,71875",Synuefai DZ-F b3-1,"-197,8125","-124,71875",-1000,"35,4",N
+2022-10-14 21:44:23,msTheo,HIP 17923,"-93,84375","-184,75","-431,84375",Pleiades Sector HB-X b1-1,"-79,53125","-204,59375","-391,46875","47,2",N
+2022-10-14 21:55:11,Bevien Irnguard,Celaeno,"-81,09375","-148,3125","-337,09375",Pleione,-77,"-146,78125","-344,125","8,3",N
+2022-10-14 22:19:44,Bevien Irnguard,Sterope II,"-81,65625","-147,28125","-340,84375",Maia,"-81,78125","-149,4375","-343,375","3,3",N
+2022-10-14 23:02:32,msTheo,Merope,"-78,59375","-149,625","-340,53125",HIP 17692,"-74,125","-143,96875","-328,78125","13,8",N
+2022-10-14 23:16:26,msTheo,Sterope II,"-81,65625","-147,28125","-340,84375",Merope,"-78,59375","-149,625","-340,53125","3,9",N
+2022-10-15 00:30:18,msTheo,Synuefe VG-A b34-4,-39,"-49,9375","-335,9375",Synuefe GX-B c16-5,"-32,9375","-57,5625","-348,15625","15,6",Y
+2022-10-15 00:54:41,msTheo,Pleiades Sector IH-V c2-2,"-73,5","-119,53125","-337,1875",Pleiades Sector HR-W d1-79,"-80,625","-146,65625","-343,25","28,7",N
+2022-10-15 05:38:11,GraphicEqualizer,California Sector HR-W d1-25,"-318,9375","-223,40625","-904,96875",California Sector EL-Y d26,"-300,6875","-234,65625","-924,59375","29,1",N
+2022-10-15 08:05:19,NeDelebreton,HIP 18154,"-64,1875","-118,53125","-292,4375",Maia,"-81,78125","-149,4375","-343,375","62,1",N
+2022-10-15 08:11:14,NeDelebreton,Maia,"-81,78125","-149,4375","-343,375",Pleiades Sector HM-V c2-5,"-45,875","-93,53125","-344,78125","66,5",N
+2022-10-15 08:55:01,kre@ture,HR 1137,"-43,9375","-109,875","-224,125",HIP 17837,"-52,15625","-130,75","-279,9375","60,2",N
+2022-10-15 14:48:17,Alton Davies,Musca Dark Region HM-V c2-35,"452,84375","3,3125","260,34375",Musca Dark Region HM-V c2-3,"441,875","5,09375","278,78125","21,5",N
+2022-10-15 16:37:47,Legs66,Taurus Dark Region JC-U b3-1,"-49,21875","-68,8125","-448,59375",Taurus Dark Region TZ-O b6-1,"-47,625","-59,78125","-398,09375","51,3",N
+2022-10-15 20:22:22,Sammi Reid,NGC 1027 Sector NP-E b12-1,"-1843,21875","82,03125","-1700,40625",NGC 1027 Sector HJ-G b11-0,"-1868,90625","85,65625","-1724,09375","35,1",N
+2022-10-15 21:43:44,Firehawk894,Pleiades Sector HR-W d1-42,"-70,1875","-150,21875","-343,84375",Pleiades Sector HW-W c1-6,"-60,15625","-159,46875","-359,375","20,7",N
+2022-10-15 22:00:08,KADOSH,Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875",Pleiades Sector IH-V c2-0,"-104,3125","-140,4375","-344,78125","4,4",N
+2022-10-16 00:23:31,Mass 1nhibited,HIP 18390,"-299,0625","-229,25","-876,125",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375","22,2",N
+2022-10-16 00:30:01,kre@ture,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Asterope,"-80,15625","-144,09375","-333,375","4,4",N
+2022-10-16 00:54:14,Mass 1nhibited,California Sector HR-W d1-28,"-329,53125","-218,5","-893,71875",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","22,1",N
+2022-10-16 01:08:41,Mass 1nhibited,California Sector GH-L b8-0,"-279,65625","-199,75","-841,8125",California Sector XU-O b6-0,"-299,46875","-204,875","-880,15625","43,5",N
+2022-10-16 01:10:58,Mass 1nhibited,California Sector XU-O b6-0,"-299,46875","-204,875","-880,15625",California Sector HR-W d1-28,"-329,53125","-218,5","-893,71875","35,7",N
+2022-10-16 02:15:31,kre@ture,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","4,4",N
+2022-10-16 02:24:42,Mass 1nhibited,California Sector GH-L b8-0,"-279,65625","-199,75","-841,8125",California Sector XU-O b6-0,"-299,46875","-204,875","-880,15625","43,5",N
+2022-10-16 02:58:04,kre@ture,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","4,4",N
+2022-10-16 03:19:28,Mallchad,Pleiades Sector ER-U b3-2,"-52,875","-77,71875","-349,375",Pleiades Sector IX-S b4-1,"-60,78125","-79,53125","-344,90625","9,3",Y
+2022-10-16 04:31:18,PanPiper,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",Y
+2022-10-16 11:01:20,Mass 1nhibited,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector IR-W d1-23,"-302,53125","-228,5625","-888,0625","32,9",N
+2022-10-16 14:25:30,Yeri-san,Pleione,-77,"-146,78125","-344,125",Atlas,"-76,71875","-147,34375","-344,4375","0,7",N
+2022-10-16 14:44:51,Yeri-san,Pleione,-77,"-146,78125","-344,125",Atlas,"-76,71875","-147,34375","-344,4375","0,7",N
+2022-10-16 14:59:25,Alfredo Cavatelli,Pleiades Sector HW-W c1-9,"-55,875","-174,5625","-373,25",Merope,"-78,59375","-149,625","-340,53125",47,N
+2022-10-16 15:03:11,Mallchad,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",Y
+2022-10-16 15:16:38,Yeri-san,Pleione,-77,"-146,78125","-344,125",Atlas,"-76,71875","-147,34375","-344,4375","0,7",N
+2022-10-16 15:20:25,Yeri-san,Pleione,-77,"-146,78125","-344,125",Atlas,"-76,71875","-147,34375","-344,4375","0,7",N
+2022-10-16 15:30:10,Yeri-san,Pleione,-77,"-146,78125","-344,125",Atlas,"-76,71875","-147,34375","-344,4375","0,7",N
+2022-10-16 15:33:23,SturmWaffel,Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875",Asterope,"-80,15625","-144,09375","-333,375","22,8",N
+2022-10-16 15:36:43,Yeri-san,Pleione,-77,"-146,78125","-344,125",Atlas,"-76,71875","-147,34375","-344,4375","0,7",N
+2022-10-16 17:15:52,Mallchad,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector ND-R b5-3,"-33,96875","-71,5625","-319,1875","38,5",Y
+2022-10-16 17:20:56,kre@ture,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","4,4",N
+2022-10-16 17:33:25,Mallchad,Pleiades Sector IH-V c2-5,"-78,71875","-138,03125","-321,78125",Celaeno,"-81,09375","-148,3125","-337,09375","18,6",N
+2022-10-16 19:28:51,BIA RoS,HR 1185,"-64,65625","-148,9375","-330,40625",Asterope,"-80,15625","-144,09375","-333,375","16,5",N
+2022-10-16 21:51:06,Gray Z7Z,Pleiades Sector MN-T c3-6,"-94,59375","-127,59375","-284,09375",Sterope II,"-81,65625","-147,28125","-340,84375","61,4",N
+2022-10-16 22:13:26,SZCZYPH,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","4,4",N
+2022-10-16 23:55:38,GrayC,HIP 17892,"-70,75","-157,9375","-352,84375",Pleione,-77,"-146,78125","-344,125","15,5",N
+2022-10-17 05:24:00,Nessy_785,Pleiades Sector TJ-Q b5-0,"-76,09375","-147,78125","-308,4375",Merope,"-78,59375","-149,625","-340,53125","32,2",N
+2022-10-17 08:03:06,LCU No Fool Like One,Pleiades Sector UZ-O b6-1,"-62,15625","-114,0625","-289,375",Pleiades Sector OI-S b4-0,"-63,1875","-135,75","-325,21875","41,9",N
+2022-10-17 08:07:50,LCU No Fool Like One,Pleiades Sector OI-S b4-0,"-63,1875","-135,75","-325,21875",Delphi,"-63,59375","-147,40625","-319,09375","13,2",N
+2022-10-17 10:24:14,Bevien Irnguard,Pleione,-77,"-146,78125","-344,125",Atlas,"-76,71875","-147,34375","-344,4375","0,7",N
+2022-10-17 10:24:52,Almus Salix,HIP 17694,"-72,25","-152,53125","-338,6875",Pleiades Sector HR-W d1-42,"-70,1875","-150,21875","-343,84375",6,
+2022-10-17 11:04:39,LilacLight,Oochoxt JS-N b12-0,"1556,9375","-183,46875","-2080,9375",Oochoxt FM-P b11-0,"1559,21875","-179,8125","-2085,78125","6,5",N
+2022-10-17 11:05:20,TheMightySardine,HIP 16302,"-154,71875","-104,5","-341,03125",Pleiades Sector IH-V c2-0,"-104,3125","-140,4375","-344,78125",62,N
+2022-10-17 11:07:43,TheMightySardine,Pleiades Sector IH-V c2-0,"-104,3125","-140,4375","-344,78125",Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875","4,4",N
+2022-10-17 11:48:09,Almus Salix,HIP 17317,"-78,03125","-166,15625","-349,09375",HIP 17694,"-72,25","-152,53125","-338,6875","18,1",
+2022-10-17 14:13:44,Almus Salix,Merope,"-78,59375","-149,625","-340,53125",Pleiades Sector HR-W d1-36,"-67,65625","-150,71875","-333,3125","13,2",
+2022-10-17 14:17:50,Almus Salix,Pleiades Sector HR-W d1-36,"-67,65625","-150,71875","-333,3125",Delphi,"-63,59375","-147,40625","-319,09375","15,2",
+2022-10-17 16:19:39,Bevien Irnguard,Pleione,-77,"-146,78125","-344,125",Atlas,"-76,71875","-147,34375","-344,4375","0,7",N
+2022-10-17 19:34:53,LilacLight,Outotch LW-O b34-0,1515,"196,75","-1601,625",Outotch NR-O b34-0,"1532,84375","175,15625","-1594,90625","28,8",N
+2022-10-17 20:37:00,kre@ture,Pleiades Sector QO-Q b5-3,"-87,65625","-141,125","-315,28125",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","19,9",N
+2022-10-17 20:51:24,LilacLight,Oochoxt EM-P b11-0,"1539,875","-182,5625","-2104,6875",Oochoxt MY-L b13-0,"1540,59375","-179,59375","-2062,3125","42,5",N
+2022-10-17 21:04:10,allan mandragoran,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector IH-V c2-17,"-91,78125","-141,0625","-340,375","13,9",N
+2022-10-17 21:14:20,SZCZYPH,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Asterope,"-80,15625","-144,09375","-333,375","4,4",N
+2022-10-17 21:50:17,Sammi Reid,Synuefai RK-V b7-0,"-684,09375","-293,1875","-897,6875",HD 16655,"-647,125","-303,1875","-893,9375","38,5",N
+2022-10-17 21:54:16,SZCZYPH,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Asterope,"-80,15625","-144,09375","-333,375","4,4",N
+2022-10-17 22:05:58,Istarias,Celaeno,"-81,09375","-148,3125","-337,09375",Merope,"-78,59375","-149,625","-340,53125","4,4",N
+2022-10-17 22:19:46,Sammi Reid,Synuefai IS-X c3-2,"-666,84375","-304,375","-894,59375",Synuefai VQ-T b8-0,"-668,8125","-302,84375","-874,1875","20,6",N
+2022-10-17 22:25:29,Sammi Reid,Synuefai EM-Z c2-0,"-684,59375","-299,34375","-906,25",Synuefai WQ-T b8-0,"-662,90625","-303,15625","-879,1875","34,9",N
+2022-10-17 22:25:30,ussnumbnuts100,HIP 22711,"-35,75","-60,84375","-366,90625",Taurus Dark Region YF-N b7-2,"-44,59375","-58,90625","-374,03125","11,5",
+2022-10-17 22:37:11,ussnumbnuts100,Pleiades Sector CQ-Y d68,"-53,0625","-75,59375","-386,59375",HIP 22460,"-41,3125","-58,96875","-354,78125","37,8",
+2022-10-17 22:40:40,ussnumbnuts100,Pleiades Sector ZZ-Y c10,"-51,3125","-79,46875","-390,46875",HIP 22460,"-41,3125","-58,96875","-354,78125","42,4",
+2022-10-17 22:44:01,ussnumbnuts100,Pleiades Sector YZ-Y c8,"-69,4375","-84,03125","-397,1875",Pleiades Sector GW-W d1-33,"-50,25","-62,8125","-326,8125",76,
+2022-10-17 23:09:30,GrayC,Merope,"-78,59375","-149,625","-340,53125",Pleiades Sector HR-W d1-74,"-87,40625","-150,90625","-344,96875","9,9",N
+2022-10-17 23:24:58,LilacLight,Oochoxt JN-N b12-0,"1519,15625","-204,3125","-2079,9375",Oochoxt MY-L b13-0,"1540,59375","-179,59375","-2062,3125","37,2",N
+2022-10-17 23:33:44,ussnumbnuts100,Hyades Sector NS-T b3-2,"-29,5625","-117,78125","-198,15625",Pleiades Sector IR-W d1-56,"-51,71875","-133,0625","-268,21875","75,1",
+2022-10-18 00:25:21,LilacLight,Oochoxt TK-I b15-0,"1534,15625","-179,75","-2016,59375",Oochoxt MY-L b13-0,"1540,59375","-179,59375","-2062,3125","46,2",N
+2022-10-18 01:23:46,LilacLight,Synuefe FI-D b18-0,"278,625","-159,5","-680,625",Synuefe FI-D b18-1,"279,03125","-164,125","-676,5625","6,2",Y
+2022-10-18 01:25:58,LilacLight,Synuefe FI-D b18-1,"279,03125","-164,125","-676,5625",Synuefe FI-D b18-0,"278,625","-159,5","-680,625","6,2",N
+2022-10-18 04:21:19,LilacLight,Synuefe JZ-Z b19-0,"236,84375","-142,875","-642,25",Synuefe QA-Y b20-1,"258,90625","-153,625","-611,125","39,6",N
+2022-10-18 04:29:47,allan mandragoran,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875","19,7",N
+2022-10-18 05:01:53,allan mandragoran,Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","19,7",N
+2022-10-18 08:00:34,LilacLight,Outotch ZE-J b37-0,"1478,84375","157,84375","-1541,15625",Outotch AN-R c18-0,"1482,28125","165,125","-1539,75","8,2",N
+2022-10-18 13:14:38,pm3,Synuefe NF-Y b20-0,"238,125","-140,34375",-621,Synuefe QA-Y b20-1,"258,90625","-153,625","-611,125","26,6",N
+2022-10-18 15:47:40,LilacLight,Oochoxt HJ-B b19-0,"1476,4375","-182,53125","-1941,1875",Oochoxt GJ-B b19-0,"1457,0625","-182,40625","-1940,46875","19,4",N
+2022-10-18 16:20:30,Sammi Reid,Outorst AZ-E b39-0,"-1622,125","80,875","-1500,0625",Outorst WD-R c18-2,"-1650,84375","79,46875","-1517,28125","33,5",N
+2022-10-18 17:08:32,Cate Nicholls,Oochoxt QR-N c9-2,"1457,25","-177,03125","-1926,1875",Oochoxt GJ-B b19-0,"1457,0625","-182,40625","-1940,46875","15,3",N
+2022-10-18 18:25:11,Seamus Donohue,Synuefe WV-T d4-9,"271,84375","-176,1875","-613,59375",Synuefe QA-Y b20-1,"258,90625","-153,625","-611,125","26,1",N
+2022-10-18 18:41:45,Epaphus,Taurus Dark Region MX-T b3-3,"-43,40625","-89,59375","-459,0625",Taurus Dark Region JH-V c2-3,"-38,78125","-74,09375","-393,65625","67,4",N
+2022-10-18 19:11:55,allan mandragoran,Pleiades Sector HR-W d1-65,"-85,28125",-145,"-339,40625",Asterope,"-80,15625","-144,09375","-333,375",8,N
+2022-10-18 19:22:18,SturmWaffel,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875","22,8",N
+2022-10-18 20:30:57,BIA RoS,HR 1185,"-64,65625","-148,9375","-330,40625",Pleiades Sector WU-O b6-2,"-47,59375","-140,40625","-285,78125","48,5",N
+2022-10-18 21:34:13,SZCZYPH,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Asterope,"-80,15625","-144,09375","-333,375","4,4",N
+2022-10-18 21:47:41,Ron Wheeler,California Sector HR-W d1-28,"-329,53125","-218,5","-893,71875",California Sector EH-L b8-0,"-320,03125","-200,09375","-842,40625","55,3",N
+2022-10-18 21:48:41,SZCZYPH,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Asterope,"-80,15625","-144,09375","-333,375","4,4",N
+2022-10-18 22:01:46,Ron Wheeler,California Sector PD-S b4-0,"-339,1875","-220,875","-924,15625",California Sector QD-S b4-0,"-320,0625","-223,34375","-921,625","19,4",N
+2022-10-18 22:46:52,Sammi Reid,Outorst GQ-N c20-2,"-1573,96875","64,46875","-1451,6875",Outorst MG-B b41-0,"-1579,09375","73,625","-1448,4375",11,N
+2022-10-18 23:44:43,Istarias,Celaeno,"-81,09375","-148,3125","-337,09375",Merope,"-78,59375","-149,625","-340,53125","4,4",N
+2022-10-19 02:29:57,Mallchad,HIP 17481,"-58,84375","-150,25","-305,53125",HR 1183,"-72,875","-145,09375","-336,96875","34,8",N
+2022-10-19 03:28:39,nerd does elite,Pleiades Sector HR-W d1-74,"-87,40625","-150,90625","-344,96875",Maia,"-81,78125","-149,4375","-343,375",6,N
+2022-10-19 04:36:10,LilacLight,Oochoxt FP-Z b19-0,"1365,6875","-169,53125","-1916,3125",Oochoxt QB-W b21-0,"1418,4375","-183,21875","-1881,625","64,6",N
+2022-10-19 10:37:53,Brooa,Col 285 Sector CF-Z c7,"-25,28125","-69,34375","-311,75",HIP 22460,"-41,3125","-58,96875","-354,78125","47,1",Y
+2022-10-19 11:16:25,Seamus Donohue,Col 285 Sector EP-Y b1-1,"-9,28125","-50,9375","-316,65625",Taurus Dark Region YF-N b7-3,"-28,96875","-55,375","-366,1875","53,5",N
+2022-10-19 12:55:47,nerd does elite,Maia,"-81,78125","-149,4375","-343,375",Pleiades Sector HR-W d1-74,"-87,40625","-150,90625","-344,96875",6,N
+2022-10-19 12:59:27,LilacLight,Oochoxt BF-I c12-0,"1343,96875","-185,53125","-1795,4375",Oochoxt DA-P b25-0,"1356,3125","-179,28125","-1800,46875","14,7",N
+2022-10-19 14:09:50,Stukjes,Synuefe MU-Z b19-0,"260,75","-164,125","-643,8125",Synuefe QA-Y b20-1,"258,90625","-153,625","-611,125","34,4",N
+2022-10-19 15:12:51,BIA RoS,Asterope,"-80,15625","-144,09375","-333,375",HR 1185,"-64,65625","-148,9375","-330,40625","16,5",N
+2022-10-19 15:31:10,Sammi Reid,Outorst FQ-N c20-2,"-1593,875","79,6875","-1443,90625",Outorst MG-B b41-0,"-1579,09375","73,625","-1448,4375","16,6",N
+2022-10-19 19:17:46,BIA RoS,Asterope,"-80,15625","-144,09375","-333,375",HR 1185,"-64,65625","-148,9375","-330,40625","16,5",N
+2022-10-19 20:26:44,Firehawk894,Pleiades Sector JC-U b3-2,"-73,625","-135,75","-359,71875",Pleiades Sector IH-V c2-5,"-78,71875","-138,03125","-321,78125","38,3",N
+2022-10-19 23:48:36,Alfredo Cavatelli,Pleiades Sector VU-O b6-3,"-71,78125","-141,375","-294,53125",Merope,"-78,59375","-149,625","-340,53125","47,2",N
+2022-10-20 02:16:33,Alfredo Cavatelli,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector IH-U b3-1,"-61,46875","-122,34375","-358,625","38,2",N
+2022-10-20 03:11:48,nerd does elite,Maia,"-81,78125","-149,4375","-343,375",Pleiades Sector HR-W d1-28,"-131,40625","-110,40625","-343,96875","63,1",N
+2022-10-20 06:54:28,Sammi Reid,Outorst ZY-V b43-0,"-1564,9375","69,875","-1386,5",Outorst ZD-W b43-0,"-1512,90625","79,5625","-1396,1875","53,8",N
+2022-10-20 09:05:31,Sammi Reid,Outorst RC-K c22-3,"-1461,09375","73,1875","-1349,625",Outorst QC-K c22-2,"-1465,5","74,65625","-1349,25","4,7",N
+2022-10-20 09:43:16,kaboom36,HIP 22460,"-41,3125","-58,96875","-354,78125",Synuefe VG-A b34-4,-39,"-49,9375","-335,9375",21,Y
+2022-10-20 10:24:15,Wotherspoon,Synuefai QC-Q b11-1,"-143,34375","-107,9375","-814,65625",Synuefai YO-M b13-0,"-139,15625","-111,46875","-783,375","31,8",N
+2022-10-20 16:18:50,ussnumbnuts100,HIP 17999,"-64,0625","-125,5","-296,71875",HIP 17704,"-72,03125","-133,90625","-311,09375","18,5",
+2022-10-20 16:20:46,Sammi Reid,NGC 752 Sector BG-W d2-4,"-831,65625","-338,5625","-1098,53125",NGC 752 Sector MJ-K b11-0,"-819,375","-339,78125","-1101,71875","12,7",Y
+2022-10-20 16:31:52,ussnumbnuts100,Maia,"-81,78125","-149,4375","-343,375",Pleiades Sector IH-V c2-8,"-75,84375","-144,875","-324,15625","20,6",
+2022-10-20 16:37:51,Pete Justice,Wregoe RA-W c15-18,"-17,28125","-19,0625","-379,65625",Synuefe LC-B a68-2,"-26,375","-28,40625","-335,59375",46,N
+2022-10-20 16:40:07,SturmWaffel,HR 1185,"-64,65625","-148,9375","-330,40625",Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875","38,9",N
+2022-10-20 18:58:28,Yeri-san,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","4,4",N
+2022-10-20 19:30:53,pokegustavo,HIP 21134,"-39,21875","-84,3125","-325,34375",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","35,4",Y
+2022-10-20 20:32:59,pokegustavo,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",Y
+2022-10-20 20:36:16,pokegustavo,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","5,2",Y
+2022-10-20 20:47:54,pokegustavo,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",Y
+2022-10-20 21:00:01,allan mandragoran,Aries Dark Region GW-W d1-35,"-54,46875","-125,15625","-248,3125",Pleiades Sector IH-V c2-7,"-78,46875","-144,9375","-324,9375","82,7",N
+2022-10-20 21:00:50,pokegustavo,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",Y
+2022-10-20 21:02:26,allan mandragoran,Pleiades Sector IH-V c2-7,"-78,46875","-144,9375","-324,9375",Asterope,"-80,15625","-144,09375","-333,375","8,6",N
+2022-10-20 21:47:47,Wotherspoon,Pleiades Sector BA-Z b1,"-73,5","-178,75",-413,Pleiades Sector QN-T b3-1,"-53,84375","-192,25","-347,8125","69,4",N
+2022-10-20 22:36:32,Sammi Reid,Outorst MW-Q b46-0,"-1485,9375","75,28125","-1328,875",Outorst QC-K c22-2,"-1465,5","74,65625","-1349,25","28,9",N
+2022-10-20 22:49:26,Ashurson,HIP 19317,"-111,40625","-111,65625","-423,34375",Pleiades Sector GW-W c1-7,"-78,25","-149,90625","-376,96875","68,7",N
+2022-10-20 23:05:33,Sammi Reid,Outorst QC-K c22-2,"-1465,5","74,65625","-1349,25",Outorst IB-F d11-10,"-1461,625","73,75","-1346,40625","4,9",Y
+2022-10-20 23:08:59,Sammi Reid,Outorst QC-K c22-2,"-1465,5","74,65625","-1349,25",Outorst IB-F d11-10,"-1461,625","73,75","-1346,40625","4,9",Y
+2022-10-21 00:07:38,Sammi Reid,Outorst IB-F d11-10,"-1461,625","73,75","-1346,40625",Outorst RC-K c22-3,"-1461,09375","73,1875","-1349,625","3,3",N
+2022-10-21 05:50:42,Sageking098,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Asterope,"-80,15625","-144,09375","-333,375","4,4",N
+2022-10-21 08:26:36,Hurix,Prooe Drye CM-P a46-2,"-2564,1875","-41,53125","709,34375",Prooe Drye CM-P a46-0,"-2562,9375","-42,34375","706,625","3,1",N
+2022-10-21 08:46:01,Hurix,Prooe Drye DM-P a46-3,"-2548,65625","-44,25","710,5625",Prooe Drye DM-P a46-1,"-2551,71875","-43,59375","712,3125","3,6",N
+2022-10-21 08:54:03,Hurix,Prooe Drye DM-P a46-0,"-2553,53125","-42,96875","707,78125",Prooe Drye DM-P a46-2,"-2550,4375","-38,59375","706,96875","5,4",Y
+2022-10-21 09:14:37,Hurix,Prooe Drye DM-P a46-3,"-2548,65625","-44,25","710,5625",Prooe Drye DM-P a46-0,"-2553,53125","-42,96875","707,78125","5,8",N
+2022-10-21 10:59:09,Sammi Reid,Synuefai AH-C b4-0,"-743,5625","-320,3125","-980,4375",Synuefai IY-Y b5-0,"-699,84375","-304,25","-939,21875","62,2",N
+2022-10-21 11:34:32,Sammi Reid,Synuefai DL-R c7-2,"-167,9375","-121,875","-742,4375",Synuefai EG-J b15-2,"-131,59375","-103,71875","-732,34375","41,9",N
+2022-10-21 12:11:20,caRGOrunner1969,HR 1183,"-72,875","-145,09375","-336,96875",Asterope,"-80,15625","-144,09375","-333,375","8,2",N
+2022-10-21 16:48:00,Yeri-san,Atlas,"-76,71875","-147,34375","-344,4375",Pleione,-77,"-146,78125","-344,125","0,7",N
+2022-10-21 17:12:21,caRGOrunner1969,HR 1183,"-72,875","-145,09375","-336,96875",Asterope,"-80,15625","-144,09375","-333,375","8,2",N
+2022-10-21 18:54:55,Yeri-san,Atlas,"-76,71875","-147,34375","-344,4375",Asterope,"-80,15625","-144,09375","-333,375",12,N
+2022-10-21 19:37:45,Sammi Reid,Synuefai GM-H b16-1,"-181,75","-100,0625","-724,71875",Synuefai EG-J b15-2,"-131,59375","-103,71875","-732,34375","50,9",N
+2022-10-21 20:05:14,MGFrank70,Pleiades Sector HR-W d1-42,"-70,1875","-150,21875","-343,84375",Delphi,"-63,59375","-147,40625","-319,09375","25,8",N
+2022-10-21 21:28:17,Istarias,Pleiades Sector IH-V c2-8,"-75,84375","-144,875","-324,15625",Merope,"-78,59375","-149,625","-340,53125","17,3",N
+2022-10-21 21:58:39,LilacLight,Prooe Drye UG-S d5-3,"-2573,96875","-55,875","705,5625",Prooe Drye DM-P a46-0,"-2553,53125","-42,96875","707,78125","24,3",N
+2022-10-21 21:59:08,Hurix,Prooe Drye AG-R a45-2,"-2544,875","-42,28125","703,3125",Prooe Drye DM-P a46-0,"-2553,53125","-42,96875","707,78125","9,8",N
+2022-10-21 22:12:38,Hurix,Prooe Drye NS-K c11-13,"-2548,1875","-48,34375","695,375",Prooe Drye DM-P a46-0,"-2553,53125","-42,96875","707,78125","14,5",N
+2022-10-21 22:25:19,Hurix,Prooe Drye RA-U d4-71,"-2483,4375","-40,78125","685,5",Prooe Drye BU-U a43-0,"-2454,34375","-43,75","677,71875","30,3",N
+2022-10-21 22:29:05,LilacLight,Prooe Drye AJ-F a38-0,"-2475,5","-42,25","621,125",Prooe Drye BU-U a43-0,"-2454,34375","-43,75","677,71875","60,4",N
+2022-10-22 00:14:49,Divji Nosi,Hyades Sector HW-W c1-14,"13,03125","-137,875","-198,65625",Aries Dark Region PN-S b4-1,"-24,6875","-143,625","-257,03125","69,7",N
+2022-10-22 00:34:30,Cate Nicholls,Prooe Drye BU-U a43-0,"-2454,34375","-43,75","677,71875",Prooe Drye SA-U d4-90,"-2456,8125","-45,4375","672,625","5,9",Y
+2022-10-22 00:48:57,Yatzil,Witch Head Sector WF-N b7-0,"339,8125","-361,21875","-659,65625",Shenve,"351,96875","-373,46875","-711,09375","54,3",N
+2022-10-22 01:07:19,kre@ture,Pleiades Sector HR-W d1-36,"-67,65625","-150,71875","-333,3125",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",17,N
+2022-10-22 04:01:32,Divji Nosi,HIP 17225,"-86,15625","-165,59375","-356,8125",Celaeno,"-81,09375","-148,3125","-337,09375","26,7",N
+2022-10-22 04:50:50,MGFrank70,Pleiades Sector PD-S b4-0,"-80,625","-156,6875","-337,4375",Maia,"-81,78125","-149,4375","-343,375","9,4",N
+2022-10-22 06:42:36,LilacLight,Oochoxt MV-Y a82-1,"2163,25","-41,09375","-1462,53125",Oochoxt TN-T a85-0,"2110,75","-42,46875","-1426,71875","63,6",N
+2022-10-22 06:56:31,Vulpes Astra,Oochoxt ZL-J d10-17,"2114,625","-35,75","-1427,65625",Oochoxt TN-T a85-0,"2110,75","-42,46875","-1426,71875","7,8",N
+2022-10-22 06:59:49,Vulpes Astra,Oochoxt TN-T a85-0,"2110,75","-42,46875","-1426,71875",Oochoxt YT-R a86-0,"2115,59375","-43,53125","-1423,34375",6,Y
+2022-10-22 07:08:58,Erion Emiroi,Oochoxt MV-Y a82-1,"2163,25","-41,09375","-1462,53125",Oochoxt TN-T a85-0,"2110,75","-42,46875","-1426,71875","63,6",N
+2022-10-22 07:19:50,Erion Emiroi,Oochoxt ZL-J d10-18,"2119,5","-35,40625","-1432,4375",Oochoxt ZL-J d10-17,"2114,625","-35,75","-1427,65625","6,8",N
+2022-10-22 07:58:30,allan mandragoran,Pleiades Sector AR-L b8-4,"-63,6875","-97,40625","-263,8125",HIP 17692,"-74,125","-143,96875","-328,78125","80,6",N
+2022-10-22 08:31:36,LilacLight,Outotch EB-F d11-20,"2047,90625","123,65625","-1312,125",Oochoxt TN-T a85-0,"2110,75","-42,46875","-1426,71875","211,4",N
+2022-10-22 09:25:56,SturmWaffel,Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875",Asterope,"-80,15625","-144,09375","-333,375","22,8",N
+2022-10-22 10:02:06,SturmWaffel,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875","22,8",N
+2022-10-22 10:48:16,SharkBiscuit,Synuefai RY-D b18-0,"-122,6875","-101,28125","-683,46875",Synuefai BV-V d3-24,"-124,3125","-96,25","-671,84375","12,8",Y
+2022-10-22 11:23:38,Hurix,HIP 22133,"-114,53125","-91,15625","-650,75",Synuefai BV-V d3-19,"-119,40625","-96,15625","-671,15625","21,6",N
+2022-10-22 12:48:35,kre@ture,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Asterope,"-80,15625","-144,09375","-333,375","4,4",N
+2022-10-22 13:19:24,Jo Quinn,Outotch DT-P c19-0,"1429,375","156,125","-1502,6875",Outotch GR-F b39-0,"1455,84375","157,3125","-1504,40625","26,6",N
+2022-10-22 13:22:20,Hurix,Outotch RU-G d10-18,"1315,46875","101,6875","-1440,125",Outotch GR-F b39-0,"1455,84375","157,3125","-1504,40625","164,1",N
+2022-10-22 14:05:48,T'Verez,Taurus Dark Region CQ-Y d45,"-27,875","-91,8125","-439,53125",Pleiades Sector GR-U b3-0,"-16,90625","-79,78125",-357,"84,1",
+2022-10-22 16:35:04,ordınary man,Synuefe QA-Y b20-1,"258,90625","-153,625","-611,125",Synuefe ZX-S b23-0,"237,3125","-144,125","-562,5",54,N
+2022-10-22 18:38:10,Nosttromo,Synuefai EG-J b15-2,"-131,59375","-103,71875","-732,34375",Synuefai BV-V d3-24,"-124,3125","-96,25","-671,84375","61,4",N
+2022-10-22 19:23:02,Yeri-san,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",Pleiades Sector IH-V c2-17,"-91,78125","-141,0625","-340,375",8,N
+2022-10-22 19:25:35,Yeri-san,Pleiades Sector IH-V c2-17,"-91,78125","-141,0625","-340,375",Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875",9,N
+2022-10-22 20:19:02,PanPiper,Pleiades Sector VZ-X b1-0,-120,"-100,1875","-399,75",Pleiades Sector VZ-X b1-1,"-116,875","-101,9375","-398,5","3,8",N
+2022-10-22 21:20:25,Sammi Reid,Synuefai BV-V d3-24,"-124,3125","-96,25","-671,84375",Synuefai BV-V d3-19,"-119,40625","-96,15625","-671,15625",5,N
+2022-10-22 21:26:44,Yeri-san,Pleiades Sector HR-W d1-65,"-85,28125",-145,"-339,40625",Pleione,-77,"-146,78125","-344,125","9,7",N
+2022-10-22 22:59:25,Wotherspoon,Pleiades Sector IX-S b4-0,"-61,25","-84,25","-341,875",HIP 22460,"-41,3125","-58,96875","-354,78125","34,7",Y
+2022-10-23 00:37:32,Gray Z7Z,Pleiades Sector KC-V c2-4,"-88,8125","-148,71875","-321,1875",Pleiades Sector IH-V c2-5,"-78,71875","-138,03125","-321,78125","14,7",N
+2022-10-23 02:06:22,XenosAurion,Pleiades Sector QD-S b4-1,"-57,21875","-149,375","-337,125",Delphi,"-63,59375","-147,40625","-319,09375","19,2",N
+2022-10-23 03:38:46,Amy Thyst,Prooe Drye YS-A b20-2,"-2371,84375","-48,40625","654,15625",Prooe Drye ZH-Y a41-1,"-2393,90625","-43,75","659,34375","23,1",N
+2022-10-23 11:35:25,Jeepin' Jenkins,Synuefe AT-S b23-2,"232,25","-147,78125","-563,71875",Synuefe GK-P b25-0,"220,4375","-140,40625","-520,46875","45,4",N
+2022-10-23 14:56:18,GrayC,HIP 17892,"-70,75","-157,9375","-352,84375",Maia,"-81,78125","-149,4375","-343,375","16,8",N
+2022-10-23 15:44:04,Andriel79,Synuefe CT-O d7-44,"-32,34375",-44,"-346,3125",HIP 22460,"-41,3125","-58,96875","-354,78125","19,4",Y
+2022-10-23 17:08:16,Iku Turso,Musca Dark Region IX-S b4-6,"443,5625","-4,46875","237,90625",Musca Dark Region CQ-Y d119,"436,6875","-8,65625","234,1875","8,9",N
+2022-10-23 17:27:10,Iku Turso,HIP 55616,"445,8125","-39,96875","202,28125",Musca Dark Region HM-V c2-18,"433,6875","1,8125","256,9375","69,9",N
+2022-10-23 17:31:01,Iku Turso,Musca Dark Region HM-V c2-18,"433,6875","1,8125","256,9375",Musca Dark Region HM-V c2-41,"428,1875","9,71875","263,53125","11,7",N
+2022-10-23 18:02:38,FULGEN,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875","8,4",Y
+2022-10-23 19:27:32,BL1P,Pleiades Sector DG-X c1-11,"-49,25","-70,4375","-360,8125",HIP 22711,"-35,75","-60,84375","-366,90625","17,6",Y
+2022-10-23 19:49:36,Elorc,Pleiades Sector RO-Q b5-1,"-78,59375","-143,09375","-320,8125",Merope,"-78,59375","-149,625","-340,53125","20,8",N
+2022-10-23 20:51:10,Sammi Reid,Synuefe ZD-R b24-1,"160,75","-138,5625","-526,71875",Synuefe KQ-N b26-1,"219,75","-138,90625","-504,875","62,9",N
+2022-10-23 21:30:25,Istarias,Merope,"-78,59375","-149,625","-340,53125",Celaeno,"-81,09375","-148,3125","-337,09375","4,4",N
+2022-10-23 23:16:22,Alfredo Cavatelli,Prooe Drye AT-A b20-0,"-2326,8125","-46,1875","639,53125",Prooe Drye ZH-Y a41-1,"-2393,90625","-43,75","659,34375",70,N
+2022-10-24 06:44:06,Carsie,Pleiades Sector PD-S b4-2,"-75,5625","-152,40625","-336,40625",Maia,"-81,78125","-149,4375","-343,375","9,8",
+2022-10-24 06:46:27,Carsie,Pleiades Sector PD-S b4-2,"-75,5625","-152,40625","-336,40625",Maia,"-81,78125","-149,4375","-343,375","9,8",
+2022-10-24 06:46:50,Carsie,Pleiades Sector PD-S b4-2,"-75,5625","-152,40625","-336,40625",Maia,"-81,78125","-149,4375","-343,375","9,8",
+2022-10-24 08:03:17,SharkBiscuit,Pleiades Sector JC-U b3-3,"-70,6875","-138,75","-358,84375",HR 1172,"-75,1875","-152,40625","-346,59375","18,9",N
+2022-10-24 10:39:32,LilacLight,Outotch QZ-G d10-10,"1423,375",171,"-1405,21875",Outotch SP-Y b42-0,"1380,3125","166,8125","-1417,96875","45,1",N
+2022-10-24 14:18:24,LilacLight,Oochorrs CJ-S b37-0,"1142,1875","-163,8125","-1534,46875",Oochorrs CC-W c18-3,"1146,5625","-177,40625","-1516,65625","22,8",N
+2022-10-24 15:29:39,SturmWaffel,Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875",Asterope,"-80,15625","-144,09375","-333,375","22,8",N
+2022-10-24 16:45:57,SturmWaffel,Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875",Asterope,"-80,15625","-144,09375","-333,375","22,8",N
+2022-10-24 16:55:28,Yeri-san,Asterope,"-80,15625","-144,09375","-333,375",Atlas,"-76,71875","-147,34375","-344,4375",12,N
+2022-10-24 16:56:06,SturmWaffel,Asterope,"-80,15625","-144,09375","-333,375",HIP 17692,"-74,125","-143,96875","-328,78125","7,6",N
+2022-10-24 17:06:21,SturmWaffel,HR 1185,"-64,65625","-148,9375","-330,40625",Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875","38,9",N
+2022-10-24 17:28:42,Yeri-san,Atlas,"-76,71875","-147,34375","-344,4375",Pleiades Sector WU-O b6-3,"-53,125","-135,0625",-288,"62,4",N
+2022-10-24 18:53:01,GrayC,Electra,"-86,0625","-159,9375","-361,65625",Pleiades Sector DL-Y d65,"-83,28125","-151,40625","-347,1875",17,N
+2022-10-24 19:28:35,Margaux Soleil,Oochoxt HB-T a99-2,"1906,4375","-43,625","-1282,0625",Oochoxt PC-Y b48-1,1921,"-49,5","-1296,1875","21,1",Y
+2022-10-24 20:19:52,StarBeaver,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","4,4",N
+2022-10-24 20:55:15,Margaux Soleil,Oochoxt HB-T a99-2,"1906,4375","-43,625","-1282,0625",Oochoxt ON-P a101-0,"1897,15625","-43,375","-1262,4375","21,7",Y
+2022-10-25 00:04:53,teeter jenkins,Prooe Drye WJ-F a38-1,"-2255,03125","-43,625","618,34375",Prooe Drye WJ-F a38-0,"-2256,3125","-44,875","618,96875","1,9",N
+2022-10-25 00:07:07,teeter jenkins,Prooe Drye WJ-F a38-0,"-2256,3125","-44,875","618,96875",Prooe Drye WJ-F a38-1,"-2255,03125","-43,625","618,34375","1,9",Y
+2022-10-25 07:20:26,SashOk50196,Col 285 Sector KP-V a4-2,"-21,21875","-42,25","-299,53125",HR 1554,"-32,375","-57,53125","-340,65625","45,3",N
+2022-10-25 07:24:02,SashOk50196,HR 1554,"-32,375","-57,53125","-340,65625",HIP 22460,"-41,3125","-58,96875","-354,78125","16,8",Y
+2022-10-25 08:29:59,MGFrank70,Asterope,"-80,15625","-144,09375","-333,375",Delphi,"-63,59375","-147,40625","-319,09375","22,1",N
+2022-10-25 10:08:31,Amy Thyst,Prooe Drye NX-I a36-1,"-2270,46875","-41,0625","598,15625",Prooe Drye WJ-F a38-0,"-2256,3125","-44,875","618,96875","25,5",N
+2022-10-25 14:55:52,jnTracks,HIP 17692,"-74,125","-143,96875","-328,78125",Pleiades Sector XF-N b7-2,"-81,6875","-124,03125","-276,71875","56,3",N
+2022-10-25 15:36:43,Mass 1nhibited,Pleiades Sector TJ-Q b5-1,"-75,4375","-160,1875","-317,34375",Pleiades Sector GR-V b2-0,"-102,25","-159,1875",-385,"72,8",N
+2022-10-25 15:49:57,mantabloke,Mel 22 Sector VO-Z c3,"-91,71875","-92,375","-558,875",Synuefai PI-M c10-1,"-96,0625","-97,71875","-597,96875","39,7",N
+2022-10-25 16:00:24,Mass 1nhibited,California Sector GH-L b8-0,"-279,65625","-199,75","-841,8125",California Sector JH-V c2-8,"-318,34375","-209,15625","-902,25","72,4",N
+2022-10-25 16:02:58,Mass 1nhibited,California Sector JH-V c2-8,"-318,34375","-209,15625","-902,25",California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875","56,8",N
+2022-10-25 16:32:37,Yeri-san,Atlas,"-76,71875","-147,34375","-344,4375",Asterope,"-80,15625","-144,09375","-333,375",12,N
+2022-10-25 17:04:38,Yeri-san,Atlas,"-76,71875","-147,34375","-344,4375",Asterope,"-80,15625","-144,09375","-333,375",12,N
+2022-10-25 17:33:33,Amy Thyst,Prooe Drye GU-R c7-5,"-2224,3125","-44,5625","574,90625",Prooe Drye XD-H a37-0,"-2212,125","-44,78125","605,3125","32,8",N
+2022-10-25 17:40:16,Mass 1nhibited,California Sector BV-Y c5,"-342,78125","-211,84375","-953,375",California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875","7,5",N
+2022-10-25 18:14:45,Amy Thyst,Prooe Drye KA-Q c8-5,"-2212,53125","-44,59375","611,03125",Prooe Drye XD-H a37-0,"-2212,125","-44,78125","605,3125","5,7",N
+2022-10-25 18:37:10,MGFrank70,Pleiades Sector HR-W d1-42,"-70,1875","-150,21875","-343,84375",Pleiades Sector GW-W c1-13,"-77,375","-156,3125","-352,03125","12,5",N
+2022-10-25 18:44:11,MGFrank70,Pleiades Sector GW-W c1-13,"-77,375","-156,3125","-352,03125",Taygeta,"-88,5","-159,6875","-366,28125","18,4",N
+2022-10-25 19:25:41,Elorc,Merope,"-78,59375","-149,625","-340,53125",HIP 17862,"-81,4375","-151,90625","-359,59375","19,4",N
+2022-10-25 20:32:56,HaLfY47,HIP 17692,"-74,125","-143,96875","-328,78125",HIP 16217,"-107,84375","-155,5625","-333,28125","35,9",N
+2022-10-25 21:08:43,GrayC,Celaeno,"-81,09375","-148,3125","-337,09375",Maia,"-81,78125","-149,4375","-343,375","6,4",N
+2022-10-25 21:20:50,Wotherspoon,Synuefai CG-V d3-14,"-516,46875","-297,125","-734,875",Synuefai WG-J b14-0,"-566,34375","-275,78125","-753,03125","57,2",N
+2022-10-25 22:12:51,GrayC,Celaeno,"-81,09375","-148,3125","-337,09375",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125","6,1",N
+2022-10-25 22:28:22,SZCZYPH,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",HIP 17692,"-74,125","-143,96875","-328,78125","11,5",N
+2022-10-26 00:19:11,Firehawk894,HIP 17704,"-72,03125","-133,90625","-311,09375",Pleiades Sector IH-V c2-5,"-78,71875","-138,03125","-321,78125","13,3",N
+2022-10-26 01:01:21,Sammi Reid,Synuefai AL-V d3-13,"-528,53125","-243,09375","-729,34375",Synuefai WG-J b14-0,"-566,34375","-275,78125","-753,03125","55,3",N
+2022-10-26 02:51:46,jellowiggler,Pleiades Sector YP-O b6-1,"-55,25","-147,625","-299,375",Pleiades Sector RO-Q b5-2,"-67,03125","-136,5625","-308,09375","18,4",N
+2022-10-26 02:55:02,jellowiggler,Pleiades Sector RO-Q b5-1,"-78,59375","-143,09375","-320,8125",HIP 17692,"-74,125","-143,96875","-328,78125","9,2",N
+2022-10-26 11:49:54,SashOk50196,Prooe Drye SZ-P a32-0,"-2064,375","-43,46875","557,8125",Prooe Drye TU-V d3-7,"-2056,3125","-46,9375","555,59375","9,1",N
+2022-10-26 15:01:27,Radiumio,Synuefe PN-B c16-13,"171,28125","-125,59375","-370,40625",Synuefe EG-D b32-0,"179,875","-119,75","-381,125","14,9",N
+2022-10-26 17:33:44,Poperrap,Pleiades Sector IR-V b2-2,"-48,8125",-153,-382,Pleiades Sector MX-T b3-2,"-60,1875","-147,59375","-357,71875","27,4",N
+2022-10-26 18:25:10,GrayC,Pleiades Sector KC-V c2-4,"-88,8125","-148,71875","-321,1875",Celaeno,"-81,09375","-148,3125","-337,09375","17,7",N
+2022-10-26 19:20:42,manakin pie-skoffa,Maia,"-81,78125","-149,4375","-343,375",Pleiades Sector MN-T c3-1,"-69,75","-131,625","-290,4375","57,1",N
+2022-10-26 19:28:43,padremo,Prooe Drye IB-X a28-2,"-1999,5625","-43,8125","523,6875",Prooe Drye TU-V d3-7,"-2056,3125","-46,9375","555,59375","65,2",N
+2022-10-26 20:15:20,Sammi Reid,Synuefai NO-L c10-0,-481,"-245,6875","-612,78125",Synuefai OO-L c10-3,"-453,1875","-240,90625","-610,46875","28,3",N
+2022-10-26 20:34:58,KENSHIRO KASUMI,Taurus Dark Region WO-A c6,"-94,34375","-86,96875","-520,5625",Mel 22 Sector VO-Z c3,"-91,71875","-92,375","-558,875","38,8",N
+2022-10-26 21:24:22,LCU No Fool Like One,Col 285 Sector CV-Y d62,"-49,15625","-33,03125","-308,21875",Synuefe QA-C b33-0,"-48,6875","-49,03125","-348,0625","42,9",N
+2022-10-26 21:26:51,LCU No Fool Like One,Synuefe QA-C b33-0,"-48,6875","-49,03125","-348,0625",HIP 22460,"-41,3125","-58,96875","-354,78125","14,1",Y
+2022-10-26 21:41:10,SZCZYPH,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Asterope,"-80,15625","-144,09375","-333,375","4,4",N
+2022-10-26 21:44:23,Istarias,Merope,"-78,59375","-149,625","-340,53125",Celaeno,"-81,09375","-148,3125","-337,09375","4,4",N
+2022-10-26 23:43:23,GrayC,Pleiades Sector IH-V c2-7,"-78,46875","-144,9375","-324,9375",Pleione,-77,"-146,78125","-344,125","19,3",N
+2022-10-27 00:11:33,Sammi Reid,Outopps UM-C b54-0,"-1260,9375","58,6875","-1181,78125",Outopps CN-B d13-16,"-1276,40625",69,"-1174,4375",20,N
+2022-10-27 00:32:22,GrayC,Merope,"-78,59375","-149,625","-340,53125",Maia,"-81,78125","-149,4375","-343,375","4,3",N
+2022-10-27 00:39:23,kre@ture,HIP 17999,"-64,0625","-125,5","-296,71875",HR 1183,"-72,875","-145,09375","-336,96875","45,6",N
+2022-10-27 13:21:40,Foperider,Prooe Drye ON-T a30-4,"-2022,09375","-43,09375","538,90625",Prooe Drye TU-V d3-7,"-2056,3125","-46,9375","555,59375","38,3",N
+2022-10-27 13:43:49,PanPiper,Taurus Dark Region DL-X b1-3,"-60,875","-87,21875","-495,59375",Taurus Dark Region WO-A c5,"-86,8125","-90,40625","-536,3125","48,4",N
+2022-10-27 14:03:23,Iku Turso,Musca Dark Region HM-M b7-9,"463,28125","-109,65625","296,28125",Musca Dark Region RU-D a13-7,"445,84375","-42,53125","293,375","69,4",N
+2022-10-27 14:53:46,PanPiper,Taurus Dark Region WO-A c3,"-84,5625","-91,625","-533,40625",Taurus Dark Region WO-A c5,"-86,8125","-90,40625","-536,3125","3,9",N
+2022-10-27 15:04:24,Vulpes Astra,HR 1185,"-64,65625","-148,9375","-330,40625",Delphi,"-63,59375","-147,40625","-319,09375","11,5",N
+2022-10-27 15:15:51,Poperrap,California Sector LC-V c2-1,"-311,5625","-234,78125","-888,5",California Sector NS-T b3-0,"-344,5625","-243,65625","-940,84375","62,5",N
+2022-10-27 15:56:46,FULGEN,California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","45,3",N
+2022-10-27 16:26:13,Seph Iridan,Pleiades Sector BM-L b8-0,"-79,15625","-123,875","-263,625",Pleiades Sector IH-V c2-12,"-85,46875","-140,4375","-322,4375","61,4",N
+2022-10-27 16:36:36,allan mandragoran,California Sector FR-M b7-0,"-296,1875","-238,5","-853,84375",HIP 18077,"-303,84375","-236,125","-860,59375","10,5",N
+2022-10-27 16:43:53,Seph Iridan,Taygeta,"-88,5","-159,6875","-366,28125",Pleiades Sector DL-Y d40,"-99,15625","-146,53125","-382,09375","23,2",N
+2022-10-27 16:44:35,Poperrap,California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875",California Sector JH-V c2-9,"-318,40625","-216,6875","-896,40625","61,2",N
+2022-10-27 17:01:44,Billaien,Col 285 Sector JQ-W b2-4,"-27,40625","-67,84375","-299,78125",Pleiades Sector ER-U b3-5,"-53,875","-81,90625","-364,3125","71,2",N
+2022-10-27 17:05:39,Yeri-san,Pleiades Sector DL-Y d67,"-80,25","-156,9375","-351,96875",Asterope,"-80,15625","-144,09375","-333,375","22,6",N
+2022-10-27 17:18:34,Wotherspoon,Synuefai ER-S b9-0,"-411,84375","-212,9375","-856,625",California Sector IH-V c2-5,"-354,5","-213,90625","-895,40625","69,2",N
+2022-10-27 17:21:18,Wotherspoon,California Sector IH-V c2-5,"-354,5","-213,90625","-895,40625",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","39,2",N
+2022-10-27 17:23:59,Poperrap,California Sector IR-W d1-19,"-270,65625","-212,65625","-840,375",California Sector KH-V c2-5,"-302,3125","-214,6875","-886,96875","56,4",N
+2022-10-27 17:30:32,Poperrap,California Sector JH-V c2-9,"-318,40625","-216,6875","-896,40625",California Sector HR-V b2-0,"-327,71875","-213,21875","-949,25","53,8",N
+2022-10-27 17:39:57,Poperrap,California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","45,3",N
+2022-10-27 17:53:42,HaLfY47,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector ON-T c3-0,"-301,71875","-224,78125","-863,3125","53,9",N
+2022-10-27 18:01:38,Wotherspoon,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector CQ-Y c5,"-350,375","-245,53125","-950,625","56,1",N
+2022-10-27 18:28:31,Epaphus,California Sector LC-V c2-7,"-336,09375","-238,5625","-902,5625",California Sector CQ-Y c5,"-350,375","-245,53125","-950,625","50,6",N
+2022-10-27 18:57:40,manakin pie-skoffa,Aries Dark Region YU-O b6-0,"-31,9375","-147,0625","-224,625",Pleiades Sector PI-T c3-9,"-57,4375","-155,375","-276,09375",58,N
+2022-10-27 19:37:54,Billaien,Taurus Dark Region BQ-Y c12,"-106,59375","-120,625","-477,46875",Taurus Dark Region WO-A c3,"-84,5625","-91,625","-533,40625","66,7",N
+2022-10-27 20:20:58,MIBE7070,California Sector WE-Q b5-0,"-320,3125","-236,84375","-887,25",HIP 18077,"-303,84375","-236,125","-860,59375","31,3",N
+2022-10-27 20:35:19,Billaien,Taurus Dark Region EW-V b2-2,"-75,5","-76,8125","-465,15625",Taurus Dark Region TZ-O b6-0,"-59,5625","-62,15625","-399,3125","69,3",N
+2022-10-27 21:08:02,Billaien,Taurus Dark Region TZ-O b6-0,"-59,5625","-62,15625","-399,3125",HIP 22460,"-41,3125","-58,96875","-354,78125","48,2",Y
+2022-10-27 21:12:20,Billaien,Pleiades Sector YZ-Y c13,"-74,78125","-100,8125","-417,3125",Pleiades Sector CW-U b3-1,"-46,53125","-62,9375","-364,5625","70,8",N
+2022-10-27 21:39:12,Poperrap,Col 285 Sector EQ-W b2-0,"-139,40625","-80,6875","-299,125",Pleiades Sector ID-R b5-2,"-143,03125","-79,34375","-324,34375","25,5",N
+2022-10-27 22:03:43,Wotherspoon,HIP 18390,"-299,0625","-229,25","-876,125",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375","22,2",N
+2022-10-27 22:26:08,Poperrap,California Sector LC-V c2-2,"-312,59375","-226,28125","-878,5",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375","12,8",N
+2022-10-27 23:06:38,Sammi Reid,Synuefe LS-Z b33-1,"159,75","-113,90625","-328,3125",Synuefe EG-D b32-0,"179,875","-119,75","-381,125","56,8",N
+2022-10-27 23:21:54,GrayC,Maia,"-81,78125","-149,4375","-343,375",HIP 17892,"-70,75","-157,9375","-352,84375","16,8",N
+2022-10-27 23:50:22,Mass 1nhibited,California Sector FB-X c1-7,"-340,15625","-211,65625","-927,9375",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","25,5",N
+2022-10-28 00:13:25,GrayC,Pleiades Sector PD-S b4-0,"-80,625","-156,6875","-337,4375",Maia,"-81,78125","-149,4375","-343,375","9,4",N
+2022-10-28 00:22:32,Mass 1nhibited,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector HR-V b2-0,"-327,71875","-213,21875","-949,25","36,8",N
+2022-10-28 00:42:58,LilacLight,Oochorrs RO-Y b47-0,"995,40625","-192,0625","-1309,6875",Oochorrs WM-L c24-2,"987,1875","-178,75","-1279,0625","34,4",N
+2022-10-28 01:02:25,GrayC,Maia,"-81,78125","-149,4375","-343,375",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",10,N
+2022-10-28 02:02:36,jellowiggler,Pleiades Sector WU-O b6-2,"-47,59375","-140,40625","-285,78125",Pleiades Sector YP-O b6-1,"-55,25","-147,625","-299,375","17,2",N
+2022-10-28 03:09:23,apsidalcolt,HIP 17692,"-74,125","-143,96875","-328,78125",HR 1185,"-64,65625","-148,9375","-330,40625","10,8",N
+2022-10-28 03:27:58,apsidalcolt,HR 1185,"-64,65625","-148,9375","-330,40625",Pleiades Sector IH-V c2-5,"-78,71875","-138,03125","-321,78125","19,8",N
+2022-10-28 09:46:35,Grimscrub,Taurus Dark Region QO-Q b5-1,"-68,96875","-65,5","-413,53125",Taurus Dark Region VB-Z a15-0,"-53,46875","-42,40625","-373,625","48,6",N
+2022-10-28 10:04:19,Grimscrub,Col 285 Sector VB-B a2-0,"-30,6875","-33,5","-329,65625",Col 285 Sector BK-Z c6,"-22,53125","-34,40625","-329,125","8,2",Y
+2022-10-28 11:54:28,Poperrap,California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","24,3",N
+2022-10-28 12:23:11,Poperrap,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector RD-S b4-0,"-302,78125","-222,1875","-920,0625","19,1",N
+2022-10-28 12:50:14,Poperrap,California Sector HW-W c1-5,"-320,875","-240,9375","-926,90625",California Sector YZ-P b5-0,"-321,5625",-253,"-903,34375","26,5",N
+2022-10-28 12:52:58,Poperrap,California Sector YZ-P b5-0,"-321,5625",-253,"-903,34375",California Sector TY-R b4-0,"-303,1875","-235,53125","-906,0625","25,5",N
+2022-10-28 13:13:14,Poperrap,California Sector FB-X c1-4,"-340,3125","-197,96875","-911,875",California Sector NI-S b4-0,"-344,3125","-201,34375","-923,25","12,5",N
+2022-10-28 13:25:22,MattW,Pleiades Sector XF-N b7-1,"-77,09375",-121,"-278,65625",HIP 17692,"-74,125","-143,96875","-328,78125","55,2",N
+2022-10-28 14:00:52,Poperrap,California Sector PD-S b4-0,"-339,1875","-220,875","-924,15625",California Sector FB-X c1-7,"-340,15625","-211,65625","-927,9375",10,N
+2022-10-28 14:52:46,Slipi,California Sector MN-T c3-2,"-380,875","-222,5625","-864,40625",California Sector PD-S b4-0,"-339,1875","-220,875","-924,15625","72,9",N
+2022-10-28 15:00:15,Foperider,Taurus Dark Region TZ-O b6-4,"-53,75","-49,65625","-387,3125",Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875",29,Y
+2022-10-28 15:06:11,manakin pie-skoffa,Pleiades Sector KS-S b4-3,"-62,90625","-91,96875","-339,21875",HIP 22460,"-41,3125","-58,96875","-354,78125","42,4",Y
+2022-10-28 15:11:40,manakin pie-skoffa,Pleiades Sector KS-S b4-3,"-62,90625","-91,96875","-339,21875",HIP 22460,"-41,3125","-58,96875","-354,78125","42,4",Y
+2022-10-28 15:26:15,GrayC,Merope,"-78,59375","-149,625","-340,53125",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",9,N
+2022-10-28 15:40:05,GrayC,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Merope,"-78,59375","-149,625","-340,53125",9,N
+2022-10-28 15:59:59,Slipi,California Sector QD-S b4-0,"-320,0625","-223,34375","-921,625",California Sector KH-V c2-0,-301,"-221,53125","-901,875","27,5",N
+2022-10-28 16:50:23,MIBE7070,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875","45,3",N
+2022-10-28 16:57:31,BIA RoS,California Sector BV-Y c5,"-342,78125","-211,84375","-953,375",California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875","7,5",N
+2022-10-28 17:35:39,Molan Ryke,Pleiades Sector LC-U b3-2,"-39,9375","-130,15625","-347,90625",HIP 22460,"-41,3125","-58,96875","-354,78125","71,5",N
+2022-10-28 17:43:08,Molan Ryke,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector JH-U b3-0,"-42,34375","-121,8125","-360,8125","63,1",Y
+2022-10-28 18:01:31,LilacLight,Outotch ZG-D d12-24,"1298,59375","134,0625","-1259,0625",Outotch QB-M b49-0,"1255,09375","139,875","-1283,9375","50,4",N
+2022-10-28 18:18:07,Ashurson,California Sector DL-Y d22,"-348,78125","-243,03125","-928,78125",California Sector TT-R b4-0,"-331,09375","-247,0625","-913,125",24,N
+2022-10-28 18:26:27,Ashurson,California Sector CQ-Y c5,"-350,375","-245,53125","-950,625",California Sector DL-Y d22,"-348,78125","-243,03125","-928,78125",22,N
+2022-10-28 20:04:12,Vegassimus,Taurus Dark Region WO-A c5,"-86,8125","-90,40625","-536,3125",Taurus Dark Region AV-Y c6,"-76,3125","-85,15625","-482,9375","54,7",N
+2022-10-28 20:13:09,Axel Silverdew,HIP 17692,"-74,125","-143,96875","-328,78125",Asterope,"-80,15625","-144,09375","-333,375","7,6",N
+2022-10-28 23:17:31,Ashurson,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector TT-R b4-0,"-331,09375","-247,0625","-913,125","32,3",N
+2022-10-29 01:54:19,Arsinoe,Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",16,N
+2022-10-29 04:27:58,Mass 1nhibited,California Sector UJ-Q b5-0,"-319,6875","-222,3125","-899,9375",California Sector HR-W d1-26,"-310,15625","-229,90625","-898,1875","12,3",N
+2022-10-29 04:47:24,Mass 1nhibited,California Sector HR-W d1-26,"-310,15625","-229,90625","-898,1875",California Sector YP-O b6-0,"-323,03125","-224,875","-881,90625","21,4",N
+2022-10-29 06:35:32,apsidalcolt,HR 1183,"-72,875","-145,09375","-336,96875",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","11,5",N
+2022-10-29 07:26:00,Mass 1nhibited,California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","24,3",N
+2022-10-29 07:51:27,Mass 1nhibited,California Sector EL-Y d22,"-303,40625","-237,75","-913,21875",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375",30,N
+2022-10-29 08:59:51,T'Verez,HR 1183,"-72,875","-145,09375","-336,96875",Asterope,"-80,15625","-144,09375","-333,375","8,2",
+2022-10-29 09:48:22,BIA RoS,California Sector BV-Y c5,"-342,78125","-211,84375","-953,375",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","46,3",N
+2022-10-29 10:17:14,Vegassimus,HIP 16158,"-11,5625","-166,1875","-211,09375",Pleiades Sector QI-T c3-3,"-14,90625","-149,8125","-274,15625","65,2",N
+2022-10-29 11:24:59,MattW,Aries Dark Region VZ-O b6-2,"-53,15625","-141,21875","-209,1875",HIP 16374,"-56,6875","-151,9375","-263,59375","55,6",N
+2022-10-29 12:22:24,T'Verez,Asterope,"-80,15625","-144,09375","-333,375",HR 1183,"-72,875","-145,09375","-336,96875","8,2",
+2022-10-29 13:26:10,KENSHIRO KASUMI,California Sector HR-W d1-28,"-329,53125","-218,5","-893,71875",California Sector EW-M b7-0,"-283,0625","-219,3125","-861,21875","56,7",N
+2022-10-29 13:53:01,badloop1,Pleiades Sector LX-U d2-42,"-93,1875","-136,3125","-260,84375",Pleiades Sector SJ-Q b5-2,"-92,78125","-153,375","-320,40625",62,N
+2022-10-29 13:55:35,badloop1,Pleiades Sector SJ-Q b5-2,"-92,78125","-153,375","-320,40625",HIP 16171,"-107,875","-155,6875","-331,65625",19,N
+2022-10-29 17:55:27,Ashurson,HD 278990,"-326,78125","-275,1875","-904,71875",HD 279128,"-314,40625","-268,25","-931,75","30,5",N
+2022-10-29 18:12:42,Ashurson,HD 279128,"-314,40625","-268,25","-931,75",California Sector SD-S b4-0,"-278,28125","-224,90625","-910,75","60,2",N
+2022-10-29 18:32:02,Sammi Reid,Synuefe EG-D b32-0,"179,875","-119,75","-381,125",Synuefe HM-B b33-2,"163,09375","-116,90625","-352,53125","33,3",N
+2022-10-29 18:57:53,Sammi Reid,Synuefe CG-D b32-2,"137,8125","-115,09375","-371,21875",Synuefe HM-B b33-2,"163,09375","-116,90625","-352,53125","31,5",N
+2022-10-29 19:05:59,Gray Z7Z,Taurus Dark Region QO-Q b5-4,"-69,15625","-76,8125","-413,1875",Pleiades Sector IX-S b4-3,"-62,0625","-84,46875","-342,3125","71,6",N
+2022-10-29 19:23:50,Sammi Reid,Synuefe LS-Z b33-1,"159,75","-113,90625","-328,3125",Synuefe HM-B b33-2,"163,09375","-116,90625","-352,53125","24,6",N
+2022-10-29 21:40:01,Gray Z7Z,Sterope II,"-81,65625","-147,28125","-340,84375",Delphi,"-63,59375","-147,40625","-319,09375","28,3",N
+2022-10-29 21:45:17,Thalnir,Taurus Dark Region OT-Q b5-0,"-81,8125","-64,625","-421,09375",Taurus Dark Region XF-N b7-3,"-51,9375","-48,8125","-374,03125","57,9",N
+2022-10-29 22:08:07,Reyedog,Taurus Dark Region KX-T b3-0,"-69,03125","-95,5","-450,6875",Taurus Dark Region AV-Y c5,"-69,90625","-89,78125","-481,0625","30,9",N
+2022-10-29 22:30:47,Tharrn,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector MC-V c2-7,"-304,71875","-231,6875","-895,8125","27,6",N
+2022-10-29 22:36:55,Tharrn,California Sector MC-V c2-7,"-304,71875","-231,6875","-895,8125",HIP 18390,"-299,0625","-229,25","-876,125","20,6",N
+2022-10-29 22:41:11,Sammi Reid,Wredguia GQ-Y c1,"-1089,3125","67,40625","-988,75",Wredguia VS-T b3-0,"-1075,78125","68,65625","-983,53125","14,6",N
+2022-10-29 22:50:35,Tharrn,California Sector TY-R b4-0,"-303,1875","-235,53125","-906,0625",California Sector EL-Y d23,"-301,4375","-231,5625","-926,96875","21,4",N
+2022-10-30 00:33:23,StarGoid,Merope,"-78,59375","-149,625","-340,53125",Pleiades Sector DL-Y d66,"-90,78125","-159,53125","-354,09375","20,8",N
+2022-10-30 01:42:12,Eeka,California Sector PD-S b4-0,"-339,1875","-220,875","-924,15625",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","22,5",N
+2022-10-30 01:44:40,CP_Luiz,Pleiades Sector FM-U b3-2,"-80,1875","-100,40625","-354,5",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",53,N
+2022-10-30 02:17:02,CP_Luiz,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","5,2",Y
+2022-10-30 02:36:55,CP_Luiz,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",Y
+2022-10-30 04:17:44,GraphicEqualizer,NGC 1514 Sector FW-W c1-2,"-249,78125","-231,375","-850,34375",California Sector IR-W d1-28,"-303,46875","-233,25","-889,5","66,5",N
+2022-10-30 05:24:17,Azicus Masrama,Synuefe HM-B b33-2,"163,09375","-116,90625","-352,53125",Synuefe LS-Z b33-3,"162,6875","-118,3125","-333,375","19,2",N
+2022-10-30 06:18:19,MIBE7070,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",HIP 17525,"-73,8125","-113,6875","-277,21875","71,6",N
+2022-10-30 08:41:57,Seamus Donohue,Col 285 Sector DP-Y b1-4,"-30,46875","-58,625","-305,90625",Pleiades Sector ER-U b3-2,"-52,875","-77,71875","-349,375","52,5",N
+2022-10-30 09:12:41,Tharrn,California Sector MX-T b3-0,"-312,4375","-211,9375","-932,59375",California Sector DL-Y d2,"-320,46875","-204,1875","-925,53125","13,2",N
+2022-10-30 09:26:12,Seamus Donohue,Synuefe HM-B b33-2,"163,09375","-116,90625","-352,53125",Synuefe LS-Z b33-1,"159,75","-113,90625","-328,3125","24,6",N
+2022-10-30 09:32:07,Tharrn,California Sector DL-Y d25,"-335,84375","-203,71875","-915,9375",California Sector DL-Y d11,"-341,625","-191,125","-907,8125","16,1",N
+2022-10-30 09:39:23,Tharrn,California Sector DL-Y d13,"-344,03125","-196,84375","-909,53125",California Sector JH-V c2-8,"-318,34375","-209,15625","-902,25","29,4",N
+2022-10-30 09:50:41,Tharrn,California Sector IR-W d1-26,"-304,53125","-202,375","-894,71875",California Sector IW-W c1-8,"-299,1875","-228,3125","-908,90625",30,N
+2022-10-30 10:55:36,LilacLight,Outotz PS-B d13-15,"1197,84375","141,3125","-1202,875",Outotz OC-F b53-0,"1180,0625",136,"-1201,71875","18,6",N
+2022-10-30 11:17:50,Reyedog,Taurus Dark Region BQ-Y d47,"-72,125","-81,84375","-484,46875",Taurus Dark Region AV-Y c6,"-76,3125","-85,15625","-482,9375","5,6",N
+2022-10-30 11:33:04,Nitrique,HIP 12099,"-101,90625","-95,46875","-165,59375",HIP 15329,"-87,84375",-52,"-167,375","45,7",N
+2022-10-30 13:06:35,don pAblo lORENZO,Synuefe PJ-W b35-2,"131,46875","-89,625","-301,4375",Synuefe LS-Z b33-1,"159,75","-113,90625","-328,3125",46,N
+2022-10-30 13:07:15,Tharrn,HIP 18458,"-305,375","-242,15625","-914,875",California Sector HW-W c1-6,"-325,59375","-236,09375","-929,1875","25,5",N
+2022-10-30 14:10:57,Mass 1nhibited,Pleiades Sector AA-Z b2,"-95,125","-183,46875","-423,25",Pleiades Sector KM-V b2-0,"-64,28125","-181,46875","-383,46875","50,4",N
+2022-10-30 14:14:10,Mass 1nhibited,HIP 17746,"-43,40625","-180,53125","-337,375",Pleiades Sector CL-O b6-1,"-8,09375","-169,0625","-303,5","50,3",N
+2022-10-30 14:17:05,AlSki,Pleiades Sector LC-V c2-1,"-61,75","-147,625","-339,09375",Merope,"-78,59375","-149,625","-340,53125",17,N
+2022-10-30 15:17:14,Galactik,Col 285 Sector DP-Y b1-3,"-36,21875","-59,6875","-311,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","43,6",Y
+2022-10-30 15:50:08,Mass 1nhibited,California Sector FH-L b8-0,"-301,75","-203,09375","-840,375",California Sector LC-V c2-2,"-312,59375","-226,28125","-878,5","45,9",N
+2022-10-30 16:12:03,Mass 1nhibited,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector DQ-Y c4,"-335,65625","-243,40625","-953,46875","50,6",N
+2022-10-30 17:33:01,Yeri-san,Pleione,-77,"-146,78125","-344,125",Atlas,"-76,71875","-147,34375","-344,4375","0,7",N
+2022-10-30 17:41:56,Yeri-san,Pleione,-77,"-146,78125","-344,125",Atlas,"-76,71875","-147,34375","-344,4375","0,7",N
+2022-10-30 19:31:45,Badboy Bellson III,Col 285 Sector GL-X c1-5,"-25,28125","-78,90625","-291,09375",Col 285 Sector CF-Z c7,"-25,28125","-69,34375","-311,75","22,8",N
+2022-10-30 19:43:05,Sammi Reid,Wredguia NL-O b6-0,"-964,03125","64,375","-920,3125",Wredguia AP-R c4-0,-940,"60,625","-862,40625","62,8",N
+2022-10-30 19:57:24,Badboy Bellson III,Pleiades Sector JX-S b4-4,"-27,125","-75,1875","-329,4375",Pleiades Sector JX-S b4-0,"-36,25","-72,90625","-341,96875","15,7",Y
+2022-10-30 20:38:06,LilacLight,Flyua Dryoae TX-X a1,"1591,53125","-44,3125","-1060,78125",Flyua Dryoae TX-X a0,"1586,71875","-43,09375","-1064,09375",6,N
+2022-10-30 20:42:26,JTZ,Pleiades Sector ZE-A d34,"-113,46875","-163,6875","-431,0625",Pleiades Sector CL-X b1-2,"-92,78125","-150,84375","-395,9375","42,7",N
+2022-10-30 21:08:09,Reyedog,Taurus Dark Region VU-O b6-4,"-51,03125","-67,5625","-400,875",Taurus Dark Region YF-N b7-2,"-44,59375","-58,90625","-374,03125","28,9",Y
+2022-10-30 21:30:09,psolem,Synuefe FR-B b33-4,"159,03125","-92,875","-349,46875",Synuefe RY-Z c16-12,"155,1875","-104,15625","-335,65625","18,2",N
+2022-10-31 00:18:26,The Shrike,98 k Tauri,"-11,6875","-50,96875","-279,53125",Synuefe GX-B c16-5,"-32,9375","-57,5625","-348,15625","72,1",
+2022-10-31 00:20:15,The Shrike,98 k Tauri,"-11,6875","-50,96875","-279,53125",Synuefe GX-B c16-5,"-32,9375","-57,5625","-348,15625","72,1",
+2022-10-31 00:40:18,Eeka,California Sector AV-Y c7,"-356,375","-217,375","-947,90625",California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875",15,N
+2022-10-31 00:49:07,psolem,Synuefe LS-Z b33-3,"162,6875","-118,3125","-333,375",Synuefe RY-Z c16-12,"155,1875","-104,15625","-335,65625","16,2",N
+2022-10-31 01:00:28,Eeka,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375","24,3",N
+2022-10-31 01:07:52,Eeka,HIP 18390,"-299,0625","-229,25","-876,125",HIP 18077,"-303,84375","-236,125","-860,59375","17,6",N
+2022-10-31 01:12:53,psolem,Synuefe RY-Z c16-11,"161,1875","-104,25","-330,8125",Synuefe LS-Z b33-1,"159,75","-113,90625","-328,3125","10,1",N
+2022-10-31 01:41:20,Gray Z7Z,Pleiades Sector HR-W d1-42,"-70,1875","-150,21875","-343,84375",Sterope II,"-81,65625","-147,28125","-340,84375","12,2",N
+2022-10-31 01:44:11,Falcon-Screech,Pleiades Sector GM-V c2-13,"-91,3125","-91,15625","-319,46875",Pleiades Sector XU-X b1-1,"-112,6875","-105,78125","-391,34375","76,4",N
+2022-10-31 01:52:46,psolem,Synuefe LS-Z b33-1,"159,75","-113,90625","-328,3125",Synuefe LS-Z b33-3,"162,6875","-118,3125","-333,375","7,3",Y
+2022-10-31 05:42:49,jellowiggler,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Atlas,"-76,71875","-147,34375","-344,4375","12,6",N
+2022-10-31 06:06:05,GraphicEqualizer,California Sector CQ-Y c5,"-350,375","-245,53125","-950,625",California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875","27,5",N
+2022-10-31 06:09:20,jellowiggler,Atlas,"-76,71875","-147,34375","-344,4375",Pleiades Sector LC-V c2-10,"-59,78125","-157,71875","-332,4375","23,2",N
+2022-10-31 06:53:33,Reyedog,HIP 20388,"-71,8125","-104,09375","-397,46875",Pleiades Sector HH-U b3-0,"-70,4375","-121,5625","-357,8125","43,4",N
+2022-10-31 06:57:41,Sageking098,Pleiades Sector MN-T c3-8,"-70,40625","-113,09375","-273,84375",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","70,2",N
+2022-10-31 07:44:36,Willyum-71,Pleiades Sector JH-V c2-4,"-60,1875","-111,46875","-318,75",Atlas,"-76,71875","-147,34375","-344,4375","47,1",N
+2022-10-31 07:53:12,Willyum-71,Atlas,"-76,71875","-147,34375","-344,4375",HIP 17862,"-81,4375","-151,90625","-359,59375","16,5",N
+2022-10-31 08:02:30,Elorc,Pleiades Sector VE-Q b5-2,"-75,65625","-175,84375","-322,125",Delphi,"-63,59375","-147,40625","-319,09375",31,N
+2022-10-31 12:23:34,Mass 1nhibited,HIP 18077,"-303,84375","-236,125","-860,59375",California Sector HR-W d1-28,"-329,53125","-218,5","-893,71875","45,5",N
+2022-10-31 13:02:01,Willyum-71,Sterope II,"-81,65625","-147,28125","-340,84375",Maia,"-81,78125","-149,4375","-343,375","3,3",N
+2022-10-31 14:01:55,Tharrn,HIP 18077,"-303,84375","-236,125","-860,59375",California Sector LC-V c2-3,"-315,40625","-228,84375","-882,59375","25,9",N
+2022-10-31 14:51:31,Foperider,Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875",HIP 22460,"-41,3125","-58,96875","-354,78125","8,4",Y
+2022-10-31 15:02:35,Foperider,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875","8,4",Y
+2022-10-31 16:18:48,Sammi Reid,Synuefai OD-Q d6-40,"-375,71875","-213,4375","-444,09375",Synuefai FS-E c14-8,"-343,65625","-211,15625","-463,75","37,7",N
+2022-10-31 17:07:46,Yeri-san,Pleiades Sector UU-O b6-2,"-98,59375","-141,09375",-293,Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875","50,2",N
+2022-10-31 17:08:39,LilacLight,Col 132 Sector XO-Z d41,"1495,9375","-47,65625","-989,8125",Col 132 Sector PD-N a13-0,"1495,96875","-43,4375","-984,78125","6,6",Y
+2022-10-31 17:17:00,daviios,HIP 16813,"-57,03125","-143,375","-268,28125",Pleiades Sector HR-W d1-36,"-67,65625","-150,71875","-333,3125","66,3",
+2022-10-31 17:18:50,daviios,HIP 16813,"-57,03125","-143,375","-268,28125",Pleiades Sector HR-W d1-36,"-67,65625","-150,71875","-333,3125","66,3",
+2022-10-31 17:20:21,LilacLight,Col 132 Sector GR-Q a11-1,"1492,59375","-42,125","-996,0625",Col 132 Sector XO-Z d41,"1495,9375","-47,65625","-989,8125",9,N
+2022-10-31 17:40:51,Yeri-san,Pleiades Sector HR-W d1-65,"-85,28125",-145,"-339,40625",Asterope,"-80,15625","-144,09375","-333,375",8,N
+2022-10-31 18:39:28,Mass 1nhibited,California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","24,3",N
+2022-10-31 19:24:54,Yeri-san,Pleiades Sector IH-V c2-17,"-91,78125","-141,0625","-340,375",Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875",9,N
+2022-10-31 20:55:00,pHILAIRONE,California Sector KH-V c2-5,"-302,3125","-214,6875","-886,96875",California Sector FB-X c1-5,"-342,4375","-202,75","-910,03125","47,8",N
+2022-10-31 21:31:16,Sammi Reid,Synuefai OD-Q d6-40,"-375,71875","-213,4375","-444,09375",Synuefai TW-F b30-0,"-314,4375","-206,875","-420,09375","66,1",N
+2022-10-31 22:06:23,Yeri-san,Pleiades Sector MN-T c3-15,"-79,53125","-144,625","-288,65625",Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875","58,5",N
+2022-10-31 22:50:43,Mass 1nhibited,California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","24,3",N
+2022-10-31 22:58:38,KENSHIRO KASUMI,California Sector FB-X c1-5,"-342,4375","-202,75","-910,03125",California Sector DL-Y d9,"-355,75","-204,09375",-943,"35,6",N
+2022-10-31 23:47:04,GrayC,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",HIP 17694,"-72,25","-152,53125","-338,6875","14,1",N
+2022-11-01 01:22:18,LilacLight,Col 132 Sector AV-X d1-30,"1407,46875","-55,71875","-982,5",Col 132 Sector BW-P b8-3,"1428,46875","-53,09375","-942,25","45,5",N
+2022-11-01 02:57:57,Arsinoe,Pleiades Sector IH-V c2-17,"-91,78125","-141,0625","-340,375",Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875",9,N
+2022-11-01 03:19:04,Ceres_O7,Pleiades Sector IX-S b4-1,"-60,78125","-79,53125","-344,90625",Pleiades Sector CG-X c1-12,"-65,0625","-101,03125","-348,53125","22,2",Y
+2022-11-01 09:38:31,SharkBiscuit,Pleiades Sector HH-U b3-2,"-66,34375","-117,28125",-364,Pleiades Sector GM-U b3-1,-51,"-101,84375","-360,90625",22,N
+2022-11-01 09:41:16,SharkBiscuit,Pleiades Sector GM-U b3-1,-51,"-101,84375","-360,90625",Pleiades Sector ER-U b3-4,"-47,375","-83,03125","-359,875","19,2",Y
+2022-11-01 09:44:21,SharkBiscuit,Pleiades Sector ER-U b3-4,"-47,375","-83,03125","-359,875",Pleiades Sector FR-U b3-0,"-38,4375",-69,"-359,96875","16,6",Y
+2022-11-01 09:48:15,SharkBiscuit,Pleiades Sector FR-U b3-0,"-38,4375",-69,"-359,96875",HIP 22460,"-41,3125","-58,96875","-354,78125","11,7",Y
+2022-11-01 09:51:03,Elorc,Pleiades Sector EL-Y d53,"-44,46875","-169,4375","-383,46875",Pleiades Sector HW-W c1-5,"-62,28125","-160,40625","-354,125","35,5",N
+2022-11-01 10:10:00,Amy Thyst,Prooe Drye MJ-C a26-2,"-1840,90625","-42,40625","487,46875",Prooe Drye TW-O b12-8,"-1835,53125","-49,65625","489,15625","9,2",N
+2022-11-01 10:24:13,SharkBiscuit,Pleiades Sector ER-U b3-2,"-52,875","-77,71875","-349,375",Pleiades Sector DG-X c1-11,"-49,25","-70,4375","-360,8125",14,Y
+2022-11-01 14:59:25,Ivard,Col 285 Sector AD-Z a2-3,"-40,40625","-43,5","-320,53125",Synuefe IR-C a67-0,"-43,84375","-43,71875","-353,03125","32,7",Y
+2022-11-01 15:00:14,Billaien,Synuefe HM-B b33-3,"163,78125","-123,75","-350,46875",Synuefe LS-Z b33-1,"159,75","-113,90625","-328,3125","24,6",N
+2022-11-01 15:14:28,Ivard,Hyades Sector VO-Z b3,"-36,125","-59,46875","-262,75",Col 285 Sector AD-Z a2-3,"-40,40625","-43,5","-320,53125","60,1",N
+2022-11-01 15:26:36,Ivard,Col 285 Sector AD-Z a2-3,"-40,40625","-43,5","-320,53125",Synuefe IR-C a67-0,"-43,84375","-43,71875","-353,03125","32,7",Y
+2022-11-01 15:51:40,Mass 1nhibited,California Sector EB-X c1-9,"-362,625","-203,4375","-927,53125",California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875",36,N
+2022-11-01 16:27:04,MIBE7070,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125","5,8",N
+2022-11-01 17:37:14,Firehawk894,HIP 17692,"-74,125","-143,96875","-328,78125",Pleiades Sector IH-V c2-5,"-78,71875","-138,03125","-321,78125","10,3",N
+2022-11-01 18:00:06,Eeka,HIP 22410,"-49,46875","-55,25","-360,59375",HIP 22460,"-41,3125","-58,96875","-354,78125","10,7",Y
+2022-11-01 18:43:44,Eeka,HIP 22460,"-41,3125","-58,96875","-354,78125",HIP 22410,"-49,46875","-55,25","-360,59375","10,7",Y
+2022-11-01 19:17:44,Eeka,HIP 22410,"-49,46875","-55,25","-360,59375",HIP 22460,"-41,3125","-58,96875","-354,78125","10,7",Y
+2022-11-01 19:26:03,Eeka,HIP 22460,"-41,3125","-58,96875","-354,78125",HIP 22410,"-49,46875","-55,25","-360,59375","10,7",Y
+2022-11-01 20:02:08,Eeka,HIP 22410,"-49,46875","-55,25","-360,59375",HIP 22460,"-41,3125","-58,96875","-354,78125","10,7",Y
+2022-11-01 20:27:00,Eeka,HIP 22460,"-41,3125","-58,96875","-354,78125",HIP 22410,"-49,46875","-55,25","-360,59375","10,7",Y
+2022-11-01 20:33:07,Mass 1nhibited,California Sector HR-V b2-0,"-327,71875","-213,21875","-949,25",California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875","16,2",N
+2022-11-01 21:24:26,Mass 1nhibited,California Sector HW-W c1-6,"-325,59375","-236,09375","-929,1875",California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875","33,4",N
+2022-11-01 21:31:39,Mass 1nhibited,California Sector MX-T b3-0,"-312,4375","-211,9375","-932,59375",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","21,1",N
+2022-11-01 22:54:55,Willyum-71,Asterope,"-80,15625","-144,09375","-333,375",Maia,"-81,78125","-149,4375","-343,375","11,5",N
+2022-11-01 23:00:30,Cate Nicholls,Pleiades Sector UT-Z b0,"-61,8125","-101,34375","-423,1875",Taurus Dark Region NI-S b4-2,"-62,34375","-83,15625","-431,75","20,1",N
+2022-11-01 23:27:33,SZCZYPH,Taurus Dark Region NI-S b4-2,"-62,34375","-83,15625","-431,75",Pleiades Sector EB-X c1-1,"-77,84375","-120,4375","-376,25","68,6",Y
+2022-11-02 00:04:35,GrayC,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",Pleione,-77,"-146,78125","-344,125","10,8",N
+2022-11-02 00:10:39,Mass 1nhibited,California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","24,3",N
+2022-11-02 00:58:42,GrayC,Pleione,-77,"-146,78125","-344,125",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125","10,8",N
+2022-11-02 01:48:11,Synngularity,California Sector LC-V c2-1,"-311,5625","-234,78125","-888,5",HIP 18077,"-303,84375","-236,125","-860,59375",29,N
+2022-11-02 02:37:14,FetalDave,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375","24,3",N
+2022-11-02 02:40:56,FetalDave,California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375",HIP 18390,"-299,0625","-229,25","-876,125","22,2",N
+2022-11-02 03:42:55,Divji Nosi,HIP 17694,"-72,25","-152,53125","-338,6875",Celaeno,"-81,09375","-148,3125","-337,09375","9,9",N
+2022-11-02 06:03:24,jellowiggler,Pleiades Sector RO-Q b5-2,"-67,03125","-136,5625","-308,09375",Pleiades Sector RO-Q b5-1,"-78,59375","-143,09375","-320,8125","18,4",N
+2022-11-02 06:06:58,jellowiggler,Pleiades Sector RO-Q b5-1,"-78,59375","-143,09375","-320,8125",HIP 17692,"-74,125","-143,96875","-328,78125","9,2",N
+2022-11-02 07:16:53,Ceres_O7,Pleiades Sector DL-Y d46,"-80,25","-179,71875","-352,125",Pleiades Sector PN-T b3-0,"-79,53125","-199,9375","-361,59375","22,3",N
+2022-11-02 10:35:21,Willyum-71,Maia,"-81,78125","-149,4375","-343,375",Asterope,"-80,15625","-144,09375","-333,375","11,5",N
+2022-11-02 12:06:06,Mass 1nhibited,California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","45,3",N
+2022-11-02 13:57:36,Elfener,California Sector CQ-Y c5,"-350,375","-245,53125","-950,625",California Sector LC-V c2-5,"-322,625","-231,71875","-897,96875","61,1",N
+2022-11-02 15:28:54,Mass 1nhibited,California Sector GR-V b2-0,"-359,34375","-220,625","-964,9375",California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875",21,N
+2022-11-02 15:51:17,Radiumio,Synuefe IZ-M d8-37,"156,125","-104,65625","-316,5625",Synuefe LS-Z b33-1,"159,75","-113,90625","-328,3125","15,4",N
+2022-11-02 17:08:31,Louis Calvert,Col 285 Sector AD-Z a2-2,"-38,5625","-44,15625","-319,25",Synuefe GX-B c16-6,"-37,25","-55,125","-347,15625",30,Y
+2022-11-02 17:15:24,Louis Calvert,Synuefe GX-B c16-6,"-37,25","-55,125","-347,15625",HIP 22460,"-41,3125","-58,96875","-354,78125","9,5",Y
+2022-11-02 17:31:29,MIBE7070,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125","5,8",N
+2022-11-02 18:57:14,SashOk50196,Col 285 Sector KP-V a4-2,"-21,21875","-42,25","-299,53125",HR 1554,"-32,375","-57,53125","-340,65625","45,3",N
+2022-11-02 19:03:05,SashOk50196,HR 1554,"-32,375","-57,53125","-340,65625",HIP 22460,"-41,3125","-58,96875","-354,78125","16,8",Y
+2022-11-02 19:48:54,SashOk50196,Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875",HIP 22460,"-41,3125","-58,96875","-354,78125","8,4",Y
+2022-11-02 19:56:41,SashOk50196,HIP 22460,"-41,3125","-58,96875","-354,78125",HIP 22711,"-35,75","-60,84375","-366,90625","13,5",Y
+2022-11-02 20:42:50,SashOk50196,Col 285 Sector AD-Z a2-3,"-40,40625","-43,5","-320,53125",Taurus Dark Region YF-N b7-3,"-28,96875","-55,375","-366,1875","48,5",Y
+2022-11-02 21:00:20,Cate Nicholls,Pleiades Sector DG-X c1-1,"-42,875","-83,46875","-384,84375",Pleiades Sector KX-S b4-3,"-20,40625","-80,6875","-338,9375","51,2",Y
+2022-11-02 21:15:33,Helmut Grokenberger,Pleiades Sector ZZ-Y c10,"-51,3125","-79,46875","-390,46875",Pleiades Sector CQ-Y d68,"-53,0625","-75,59375","-386,59375","5,8",Y
+2022-11-02 22:38:44,Mass 1nhibited,California Sector GR-V b2-0,"-359,34375","-220,625","-964,9375",California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875",21,N
+2022-11-02 22:51:15,Wegian,Col 285 Sector MV-T a5-2,"-43,28125","-44,8125","-287,75",Synuefe VG-A b34-4,-39,"-49,9375","-335,9375","48,6",N
+2022-11-02 22:58:41,Amy Thyst,Prooe Drye PI-Z d1-25,"-1671,46875","-54,84375","412,84375",Prooe Drye QI-Z d1-43,"-1660,46875","-51,25","433,46875","23,6",N
+2022-11-02 23:05:36,Nathan Kerbonaut,HIP 22460,"-41,3125","-58,96875","-354,78125",Synuefe RA-C b33-0,"-25,875","-50,5","-364,125","19,9",Y
+2022-11-02 23:07:38,Wegian,Col 285 Sector DP-Y b1-1,"-41,40625","-60,40625","-319,21875",HIP 22460,"-41,3125","-58,96875","-354,78125","35,6",Y
+2022-11-02 23:16:32,Wegian,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector ZZ-Y c10,"-51,3125","-79,46875","-390,46875","42,4",Y
+2022-11-02 23:32:02,Wegian,Taurus Dark Region QO-Q b5-4,"-69,15625","-76,8125","-413,1875",Pleiades Sector DG-X c1-12,"-52,5","-70,6875","-365,75","50,6",N
+2022-11-02 23:33:36,Chase Hutchins,Col 285 Sector RB-S a6-1,"-30,875","-44,65625","-284,96875",Pleiades Sector HC-T b4-0,"-43,25","-60,03125","-343,03125","61,3",N
+2022-11-02 23:35:06,Wegian,Pleiades Sector DG-X c1-12,"-52,5","-70,6875","-365,75",Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875","12,9",Y
+2022-11-02 23:37:07,Chase Hutchins,Pleiades Sector HC-T b4-0,"-43,25","-60,03125","-343,03125",HIP 22460,"-41,3125","-58,96875","-354,78125",12,Y
+2022-11-02 23:59:22,Reyedog,Pleiades Sector HR-W d1-70,"-101,5","-181,375","-266,625",Pleiades Sector OI-T c3-6,"-80,65625","-151,625","-292,375","44,5",N
+2022-11-03 00:04:42,Reyedog,Pleiades Sector OI-T c3-6,"-80,65625","-151,625","-292,375",Delphi,"-63,59375","-147,40625","-319,09375",32,N
+2022-11-03 00:34:22,Louis Calvert,Synuefe GX-B c16-6,"-37,25","-55,125","-347,15625",HR 1490,"-49,5625","-73,125","-371,125","32,4",Y
+2022-11-03 00:49:45,LBBP,Pleiades Sector XE-Y b1-4,"-42,6875","-81,5","-398,78125",Pleiades Sector ZZ-Y c10,"-51,3125","-79,46875","-390,46875","12,1",Y
+2022-11-03 01:36:08,Chase Hutchins,Pleiades Sector HC-T b4-0,"-43,25","-60,03125","-343,03125",HIP 22460,"-41,3125","-58,96875","-354,78125",12,Y
+2022-11-03 02:05:42,wavelengthinversion,Pleiades Sector QD-S b4-1,"-57,21875","-149,375","-337,125",HR 1183,"-72,875","-145,09375","-336,96875","16,2",
+2022-11-03 02:32:44,wavelengthinversion,Delphi,"-63,59375","-147,40625","-319,09375",HIP 18266,"-51,40625","-135,65625","-304,125","22,6",
+2022-11-03 06:40:37,GraphicEqualizer,Synuefai BO-C b32-2,"-280,75",-193,"-384,9375",Synuefai RB-G b30-1,"-308,46875","-202,125","-422,25","47,4",N
+2022-11-03 07:52:35,PanPiper,Pleiades Sector DG-X c1-13,"-49,46875","-83,21875","-376,0625",HIP 19604,"-57,40625","-123,90625",-363,"43,5",Y
+2022-11-03 09:30:22,Ceres_O7,Pleiades Sector QY-R b4-1,"-93,625","-175,9375","-341,25",Pleiades Sector PD-S b4-1,"-82,0625","-155,71875","-336,46875","23,8",N
+2022-11-03 09:38:00,SturmWaffel,Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875",HIP 18154,"-64,1875","-118,53125","-292,4375","65,8",N
+2022-11-03 11:22:54,SashOk50196,Merope,"-78,59375","-149,625","-340,53125",HIP 19176,"-37,40625","-134,875","-324,0625","46,7",N
+2022-11-03 11:36:01,SashOk50196,Synuefe LS-Z b33-1,"159,75","-113,90625","-328,3125",Synuefe IZ-M d8-37,"156,125","-104,65625","-316,5625","15,4",N
+2022-11-03 11:49:47,Louis Calvert,Pleiades Sector CG-W b2-2,"-53,59375","-102,3125","-383,3125",Pleiades Sector ZZ-Y c10,"-51,3125","-79,46875","-390,46875",24,N
+2022-11-03 12:11:34,Willyum-71,Maia,"-81,78125","-149,4375","-343,375",Asterope,"-80,15625","-144,09375","-333,375","11,5",N
+2022-11-03 13:15:08,Willyum-71,Maia,"-81,78125","-149,4375","-343,375",HIP 16996,"-85,625","-147,28125","-321,71875","22,1",N
+2022-11-03 20:59:15,Cate Nicholls,Pleiades Sector CQ-Y d68,"-53,0625","-75,59375","-386,59375",Pleiades Sector ZZ-Y c10,"-51,3125","-79,46875","-390,46875","5,8",Y
+2022-11-03 21:39:17,ALZAROC,Hyades Sector DG-D a1,"-32,5","-29,125","-276,28125",Synuefe VG-A b34-2,"-34,4375","-47,65625","-335,96875","62,5",N
+2022-11-03 22:22:50,SZCZYPH,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Pleiades Sector MN-T c3-10,"-87,78125","-131,03125","-267,0625","68,9",N
+2022-11-04 00:26:40,Bommy,Pleiades Sector OI-S b4-0,"-63,1875","-135,75","-325,21875",Atlas,"-76,71875","-147,34375","-344,4375","26,2",N
+2022-11-04 05:52:54,Willyum-71,HIP 16996,"-85,625","-147,28125","-321,71875",Asterope,"-80,15625","-144,09375","-333,375","13,3",N
+2022-11-04 06:07:09,Willyum-71,Asterope,"-80,15625","-144,09375","-333,375",HIP 16996,"-85,625","-147,28125","-321,71875","13,3",N
+2022-11-04 12:13:41,Amy Thyst,Prooe Drye EH-S a17-0,"-1560,40625",-44,"404,90625",Prooe Drye RY-V b8-6,"-1557,1875","-51,75","401,53125",9,N
+2022-11-04 12:43:42,Sammi Reid,Synuefe LS-Z b33-1,"159,75","-113,90625","-328,3125",Synuefe IZ-M d8-37,"156,125","-104,65625","-316,5625","15,4",N
+2022-11-04 13:08:45,Sammi Reid,Wredguia AP-R c4-0,-940,"60,625","-862,40625",Wredguia GK-H b10-1,"-904,15625","57,0625","-831,625","47,4",N
+2022-11-04 13:26:37,Mass 1nhibited,California Sector GR-V b2-0,"-359,34375","-220,625","-964,9375",California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875",21,N
+2022-11-04 15:02:11,MIBE7070,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","5,8",N
+2022-11-04 16:56:14,Flaw less,Pleiades Sector FW-W d1-75,"-65,84375","-83,71875","-327,78125",Pleiades Sector GW-W d1-33,"-50,25","-62,8125","-326,8125","26,1",Y
+2022-11-04 17:07:03,Mass 1nhibited,California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","45,3",N
+2022-11-04 17:18:45,Sammi Reid,Wredguia HV-P c5-5,-812,"80,3125","-789,78125",Wredguia KB-O c6-6,"-833,375","65,46875","-765,9375","35,3",N
+2022-11-04 18:33:10,Mass 1nhibited,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector HR-W d1-26,"-310,15625","-229,90625","-898,1875","22,4",N
+2022-11-04 18:35:21,Mass 1nhibited,California Sector HR-W d1-26,"-310,15625","-229,90625","-898,1875",California Sector IR-W d1-23,"-302,53125","-228,5625","-888,0625","12,7",N
+2022-11-04 18:52:19,Helmut Grokenberger,Pleione,-77,"-146,78125","-344,125",Pleiades Sector MN-T c3-1,"-69,75","-131,625","-290,4375","56,3",N
+2022-11-04 19:22:02,TheProRockPL,Synuefai OE-B c16-10,"-269,4375","-187,96875","-383,71875",Synuefai BO-C b32-2,"-280,75",-193,"-384,9375","12,4",N
+2022-11-04 19:27:01,Sammi Reid,Wredguia FP-Y b14-0,"-801,65625","55,46875","-742,71875",Wredguia AJ-A b14-1,"-823,8125","62,125","-755,03125","26,2",N
+2022-11-04 19:57:29,Talisaerin,Synuefe WK-U b36-2,"145,25","-105,75","-284,1875",Synuefe IZ-M d8-37,"156,125","-104,65625","-316,5625","34,2",N
+2022-11-04 20:55:34,Sammi Reid,Synuefai BO-C b32-2,"-280,75",-193,"-384,9375",Synuefai BO-C b32-1,"-278,125","-191,28125","-380,21875","5,7",Y
+2022-11-04 20:56:56,Ter0k,Synuefe VE-Y c17-18,"148,8125","-89,875","-288,3125",Synuefe IZ-M d8-37,"156,125","-104,65625","-316,5625","32,7",N
+2022-11-04 21:09:15,Sammi Reid,Synuefai BO-C b32-1,"-278,125","-191,28125","-380,21875",Synuefai KY-C c15-3,"-272,25","-189,71875","-392,09375","13,3",N
+2022-11-04 21:32:34,Ter0k,Wregoe TQ-Z b0,"1039,15625","130,15625","-1037,625",Outotz II-U b58-0,"1060,0625","121,78125","-1077,25","45,6",N
+2022-11-04 21:47:06,ndrv,HIP 21760,"-42,8125","-51,03125","-276,46875",Pleiades Sector FR-V c2-1,"-58,125","-60,59375","-343,9375","69,8",N
+2022-11-04 22:00:41,ndrv,Taurus Dark Region NI-S b4-2,"-62,34375","-83,15625","-431,75",Pleiades Sector ZZ-Y c10,"-51,3125","-79,46875","-390,46875","42,9",N
+2022-11-04 22:14:24,Amy Thyst,Prooe Drye HW-Y c3-12,"-1531,71875","-53,5625","377,1875",Prooe Drye HW-Y c3-7,"-1514,25","-53,375","389,40625","21,3",N
+2022-11-04 22:17:22,ndrv,Pleiades Sector ZZ-Y c10,"-51,3125","-79,46875","-390,46875",Pleiades Sector LI-R b5-0,"-42,9375","-63,34375","-322,40625","70,4",Y
+2022-11-04 22:48:46,Sammi Reid,Synuefai KY-C c15-3,"-272,25","-189,71875","-392,09375",Synuefai BO-C b32-1,"-278,125","-191,28125","-380,21875","13,3",N
+2022-11-04 22:50:43,Willyum-71,HIP 15823,"-69,5","-209,1875","-326,5",Pleiades Sector VE-Q b5-2,"-75,65625","-175,84375","-322,125","34,2",N
+2022-11-04 23:12:20,Bannini,Witch Head Sector EB-X c1-0,"358,46875","-359,375","-729,46875",Shenve,"351,96875","-373,46875","-711,09375","24,1",N
+2022-11-04 23:26:39,BlakeA,Pleiades Sector IH-V c2-5,"-78,71875","-138,03125","-321,78125",Celaeno,"-81,09375","-148,3125","-337,09375","18,6",
+2022-11-05 00:50:02,Mass 1nhibited,California Sector MX-T b3-0,"-312,4375","-211,9375","-932,59375",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","21,1",N
+2022-11-05 00:53:38,Zulu Romeo,Col 285 Sector FQ-X c1-5,"2,25","-57,3125","-286,125",HR 1554,"-32,375","-57,53125","-340,65625","64,6",N
+2022-11-05 01:06:00,BlakeA,Pleiades Sector IH-V c2-5,"-78,71875","-138,03125","-321,78125",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125","21,2",
+2022-11-05 01:20:51,BlakeA,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",Merope,"-78,59375","-149,625","-340,53125","8,5",
+2022-11-05 08:55:15,MIBE7070,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","5,8",N
+2022-11-05 10:45:19,Mass 1nhibited,California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","24,3",N
+2022-11-05 11:56:09,Mass 1nhibited,California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","24,3",N
+2022-11-05 14:12:42,Sammi Reid,Pleiades Sector ZZ-Y c10,"-51,3125","-79,46875","-390,46875",Pleiades Sector AL-W b2-0,"-46,03125","-79,4375","-370,34375","20,8",Y
+2022-11-05 15:09:53,Yeri-san,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Asterope,"-80,15625","-144,09375","-333,375","4,4",N
+2022-11-05 16:37:51,Alton Davies,Pleiades Sector IR-W c1-5,"-86,4375","-214,46875",-362,HIP 17000,"-84,65625","-171,75","-353,125","43,7",N
+2022-11-05 16:50:20,Alton Davies,HIP 17000,"-84,65625","-171,75","-353,125",Electra,"-86,0625","-159,9375","-361,65625","14,6",N
+2022-11-05 16:54:27,LilacLight,Col 132 Sector OA-H b13-3,"1284,40625","-55,59375","-838,65625",Col 132 Sector OA-H b13-1,"1282,25","-54,53125","-839,53125","2,6",N
+2022-11-05 17:27:45,LilacLight,Col 132 Sector TM-D b15-0,"1215,03125","-49,0625","-790,65625",Col 132 Sector TM-D b15-1,"1215,03125","-54,03125","-788,625","5,4",N
+2022-11-05 17:38:45,LilacLight,Col 132 Sector JA-H b13-1,"1186,3125","-53,34375","-835,875",Col 132 Sector TM-D b15-1,"1215,03125","-54,03125","-788,625","55,3",N
+2022-11-05 20:25:24,ENERGYMR,Pleione,-77,"-146,78125","-344,125",Celaeno,"-81,09375","-148,3125","-337,09375","8,3",N
+2022-11-05 21:05:18,LilacLight,Col 132 Sector QF-L c9-10,"1148,3125","-54,4375","-741,40625",Col 132 Sector QF-L c9-8,"1150,375",-55,"-744,40625","3,7",N
+2022-11-05 21:33:43,Mass 1nhibited,California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","24,3",N
+2022-11-05 22:37:24,Mass 1nhibited,HIP 18077,"-303,84375","-236,125","-860,59375",California Sector HR-W d1-28,"-329,53125","-218,5","-893,71875","45,5",N
+2022-11-05 22:42:20,Mass 1nhibited,California Sector HR-W d1-28,"-329,53125","-218,5","-893,71875",California Sector FB-X c1-8,"-342,90625","-208,34375","-932,3125","42,1",N
+2022-11-05 23:02:39,Sammi Reid,Pleiades Sector ZK-W b2-2,"-68,59375","-73,78125","-383,4375",Mel 22 Sector KY-F b12-0,-5,"-83,03125","-361,1875",68,Y
+2022-11-05 23:09:40,Mass 1nhibited,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector NS-T b3-0,"-344,5625","-243,65625","-940,84375","45,7",N
+2022-11-05 23:20:54,Stefanos,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","4,4",N
+2022-11-05 23:22:55,LilacLight,NGC 2232 Sector BL-N b7-0,"716,34375","-164,46875","-962,90625",NGC 2232 Sector IM-L b8-0,"735,90625","-175,875","-944,96875","28,9",N
+2022-11-05 23:36:10,Mass 1nhibited,California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","45,3",N
+2022-11-06 00:06:52,Stefanos,Hyades Sector DB-X c1-3,"-86,15625","-86,75","-215,0625",Pleiades Sector BM-L b8-1,"-81,9375","-123,8125","-264,15625","61,7",N
+2022-11-06 00:13:46,Stefanos,Pleiades Sector BM-L b8-1,"-81,9375","-123,8125","-264,15625",Pleiades Sector IH-V c2-6,"-77,625","-142,21875","-325,25",64,N
+2022-11-06 00:27:02,Mass 1nhibited,California Sector GR-V b2-0,"-359,34375","-220,625","-964,9375",California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875",21,N
+2022-11-06 01:43:25,Gray Z7Z,Sterope II,"-81,65625","-147,28125","-340,84375",Merope,"-78,59375","-149,625","-340,53125","3,9",N
+2022-11-06 01:53:54,Mass 1nhibited,California Sector HW-W c1-4,"-330,71875","-233,59375","-909,1875",California Sector HR-W d1-28,"-329,53125","-218,5","-893,71875","21,6",N
+2022-11-06 02:13:24,Mass 1nhibited,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector NS-T b3-0,"-344,5625","-243,65625","-940,84375","45,7",N
+2022-11-06 02:35:30,Mass 1nhibited,California Sector HW-W c1-0,"-341,09375","-261,21875","-942,5",California Sector HW-W c1-4,"-330,71875","-233,59375","-909,1875","44,5",N
+2022-11-06 03:44:15,TomCatT,Asterope,"-80,15625","-144,09375","-333,375",Maia,"-81,78125","-149,4375","-343,375","11,5",N
+2022-11-06 03:44:38,Mass 1nhibited,HIP 18077,"-303,84375","-236,125","-860,59375",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375","34,7",N
+2022-11-06 04:21:16,Mass 1nhibited,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector ZP-O b6-0,"-290,84375","-214,0625","-881,03125","43,6",N
+2022-11-06 04:36:54,BlakeA,HIP 17837,"-52,15625","-130,75","-279,9375",HIP 17692,"-74,125","-143,96875","-328,78125","55,2",
+2022-11-06 08:16:27,TomCatT,Asterope,"-80,15625","-144,09375","-333,375",Maia,"-81,78125","-149,4375","-343,375","11,5",N
+2022-11-06 08:18:04,LilacLight,Wregoe UL-Z b0,"1031,375","113,1875","-1042,59375",Wregoe QF-B b0,"1031,15625","109,28125","-1048,6875","7,2",Y
+2022-11-06 09:27:36,msTheo,Musca Dark Region BQ-Y d172,"382,28125","-0,25","225,34375",Musca Dark Region YB-I a11-0,"417,46875","-2,28125","265,1875","53,2",N
+2022-11-06 11:08:29,FULGEN,Pleiades Sector CW-U b3-1,"-46,53125","-62,9375","-364,5625",HIP 22460,"-41,3125","-58,96875","-354,78125","11,8",Y
+2022-11-06 11:11:33,FULGEN,Pleiades Sector CW-U b3-1,"-46,53125","-62,9375","-364,5625",HIP 22460,"-41,3125","-58,96875","-354,78125","11,8",Y
+2022-11-06 11:20:22,TomCatT,Asterope,"-80,15625","-144,09375","-333,375",Maia,"-81,78125","-149,4375","-343,375","11,5",N
+2022-11-06 12:07:00,Azicus Masrama,Musca Dark Region PJ-P b6-11,426,"1,28125","290,21875",Musca Dark Region PJ-P b6-1,"432,625","2,53125","288,6875","6,9",N
+2022-11-06 14:43:53,BlakeA,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",Pleiades Sector OI-T c3-6,"-80,65625","-151,625","-292,375","47,5",
+2022-11-06 15:03:28,Firedrax,HR 1183,"-72,875","-145,09375","-336,96875",Pleiades Sector YP-O b6-2,"-58,15625","-146,75","-303,1875","36,9",N
+2022-11-06 15:44:21,Sammi Reid,Synuefe HZ-M d8-68,"43,375","-89,21875","-341,875",Pleiades Sector GR-U b3-2,"-20,21875","-76,4375","-361,15625","67,7",N
+2022-11-06 15:46:38,Sammi Reid,Pleiades Sector GR-U b3-2,"-20,21875","-76,4375","-361,15625",Pleiades Sector ZK-W b2-2,"-68,59375","-73,78125","-383,4375","53,3",Y
+2022-11-06 17:46:42,PanPiper,Pleiades Sector DG-X c1-3,"-48,8125","-92,59375","-377,71875",Pleiades Sector DG-X c1-14,"-45,625","-80,78125","-376,4375","12,3",Y
+2022-11-06 17:50:18,Karl berg,Arietis Sector ZE-A d75,"-87,15625","-99,46875","-197,40625",Arietis Sector BL-X b1-5,"-87,46875","-54,96875","-173,40625","50,6",N
+2022-11-06 18:07:09,Wotherspoon,HIP 22410,"-49,46875","-55,25","-360,59375",HIP 22460,"-41,3125","-58,96875","-354,78125","10,7",Y
+2022-11-06 18:09:24,Wotherspoon,HIP 22410,"-49,46875","-55,25","-360,59375",HIP 22460,"-41,3125","-58,96875","-354,78125","10,7",Y
+2022-11-06 18:38:56,Mass 1nhibited,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector ZP-O b6-0,"-290,84375","-214,0625","-881,03125","43,6",N
+2022-11-06 18:48:12,Wotherspoon,HIP 22410,"-49,46875","-55,25","-360,59375",HIP 22460,"-41,3125","-58,96875","-354,78125","10,7",Y
+2022-11-06 19:06:33,Wotherspoon,HIP 22460,"-41,3125","-58,96875","-354,78125",HIP 22410,"-49,46875","-55,25","-360,59375","10,7",Y
+2022-11-06 19:28:42,Mass 1nhibited,California Sector EL-Y d22,"-303,40625","-237,75","-913,21875",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375",30,N
+2022-11-06 19:50:19,Sammi Reid,Synuefe LY-X b34-1,"79,03125","-124,03125","-319,65625",Synuefe OY-X b34-3,"144,03125","-112,25","-320,15625","66,1",N
+2022-11-06 20:02:57,Mass 1nhibited,California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","24,3",N
+2022-11-06 20:09:57,Mass 1nhibited,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector ZP-O b6-0,"-290,84375","-214,0625","-881,03125","43,6",N
+2022-11-06 20:20:03,Mass 1nhibited,HIP 18390,"-299,0625","-229,25","-876,125",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375","22,2",N
+2022-11-06 21:31:16,Sammi Reid,Pleiades Sector ZK-W b2-2,"-68,59375","-73,78125","-383,4375",Pleiades Sector ZZ-Y c10,"-51,3125","-79,46875","-390,46875","19,5",Y
+2022-11-06 21:35:59,Sammi Reid,Pleiades Sector ZZ-Y c10,"-51,3125","-79,46875","-390,46875",Pleiades Sector AL-W b2-0,"-46,03125","-79,4375","-370,34375","20,8",Y
+2022-11-06 21:43:33,Sammi Reid,Taurus Dark Region BB-N b7-4,"-19,40625","-80,84375","-380,96875",Pleiades Sector AL-W b2-0,"-46,03125","-79,4375","-370,34375","28,7",Y
+2022-11-06 22:48:31,LilacLight,Col 132 Sector WY-Z b16-3,"1124,4375","-51,0625","-749,9375",Col 132 Sector QF-L c9-8,"1150,375",-55,"-744,40625","26,8",N
+2022-11-06 23:02:34,MGFrank70,Pleione,-77,"-146,78125","-344,125",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","12,1",N
+2022-11-07 00:15:41,Gray Z7Z,Merope,"-78,59375","-149,625","-340,53125",Pleione,-77,"-146,78125","-344,125","4,9",N
+2022-11-07 00:29:06,Gray Z7Z,Pleione,-77,"-146,78125","-344,125",Merope,"-78,59375","-149,625","-340,53125","4,9",N
+2022-11-07 01:32:38,Sammi Reid,Taurus Dark Region BB-N b7-4,"-19,40625","-80,84375","-380,96875",Pleiades Sector AL-W b2-0,"-46,03125","-79,4375","-370,34375","28,7",Y
+2022-11-07 01:53:56,GrayC,Celaeno,"-81,09375","-148,3125","-337,09375",Maia,"-81,78125","-149,4375","-343,375","6,4",N
+2022-11-07 04:05:21,Mass 1nhibited,California Sector MH-V b2-0,"-317,15625","-251,96875","-956,28125",California Sector EL-Y d22,"-303,40625","-237,75","-913,21875","47,4",N
+2022-11-07 04:07:50,Mass 1nhibited,California Sector EL-Y d22,"-303,40625","-237,75","-913,21875",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375",30,N
+2022-11-07 04:55:42,Mass 1nhibited,California Sector HW-W c1-0,"-341,09375","-261,21875","-942,5",California Sector HW-W c1-4,"-330,71875","-233,59375","-909,1875","44,5",N
+2022-11-07 05:33:41,KnightArch,Pleiades Sector CQ-Y d60,"-46,21875","-83,5",-367,Pleiades Sector ZZ-Y c10,"-51,3125","-79,46875","-390,46875","24,4",Y
+2022-11-07 05:54:21,Mass 1nhibited,California Sector MH-V b2-0,"-317,15625","-251,96875","-956,28125",California Sector IW-W c1-5,"-303,15625","-246,3125","-912,21875","46,6",N
+2022-11-07 06:13:46,Mass 1nhibited,California Sector UJ-Q b5-0,"-319,6875","-222,3125","-899,9375",California Sector FB-X c1-8,"-342,90625","-208,34375","-932,3125","42,2",N
+2022-11-07 07:22:18,KnightArch,Pleiades Sector AL-W b2-0,"-46,03125","-79,4375","-370,34375",HIP 22711,"-35,75","-60,84375","-366,90625","21,5",Y
+2022-11-07 07:35:10,lupus stellaris,Col 132 Sector QF-L c9-9,"1146,84375","-53,90625","-742,40625",Col 132 Sector QF-L c9-6,"1158,84375","-55,9375","-740,25","12,4",N
+2022-11-07 07:44:35,lupus stellaris,Col 132 Sector QF-L c9-6,"1158,84375","-55,9375","-740,25",Col 132 Sector XY-Z b16-1,"1147,09375","-57,9375","-748,1875","14,3",N
+2022-11-07 08:43:56,KnightArch,HIP 22711,"-35,75","-60,84375","-366,90625",HIP 22460,"-41,3125","-58,96875","-354,78125","13,5",Y
+2022-11-07 10:44:54,lupus stellaris,Col 132 Sector QF-L c9-8,"1150,375",-55,"-744,40625",Col 132 Sector MZ-M c8-4,"1158,90625","-55,96875","-746,59375","8,9",Y
+2022-11-07 11:09:46,HAyden eliback,Synuefe XV-S b37-5,"133,4375","-85,8125","-262,8125",Synuefe UP-U b36-3,"144,65625","-95,84375","-269,625","16,5",N
+2022-11-07 15:29:13,Mass 1nhibited,California Sector MX-T b3-0,"-312,4375","-211,9375","-932,59375",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","21,1",N
+2022-11-07 18:39:23,MintCondition,Pleiades Sector LS-T c3-15,"-57,59375","-72,0625","-301,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","57,2",N
+2022-11-07 21:12:16,Amy Thyst,Prooe Drye PC-B d1-72,"-1382,78125","-57,375","336,53125",Prooe Drye HQ-A c3-11,"-1383,84375","-52,96875","348,25","12,6",N
+2022-11-07 21:24:03,Talisaerin,Synuefe VE-Y c17-20,"147,65625","-100,96875","-295,53125",Synuefe UP-U b36-3,"144,65625","-95,84375","-269,625","26,6",N
+2022-11-07 21:42:50,Amy Thyst,Swoiwns AS-E b4-1,"-1260,125","-48,375","310,6875",Swoiwns AS-E b4-7,"-1245,8125","-53,90625","307,71875","15,6",N
+2022-11-07 21:50:00,Sammi Reid,Taurus Dark Region BB-N b7-4,"-19,40625","-80,84375","-380,96875",Pleiades Sector AL-W b2-0,"-46,03125","-79,4375","-370,34375","28,7",Y
+2022-11-07 22:31:22,jellowiggler,HIP 17692,"-74,125","-143,96875","-328,78125",Maia,"-81,78125","-149,4375","-343,375","17,4",N
+2022-11-07 23:25:35,Stefanos,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Asterope,"-80,15625","-144,09375","-333,375","4,4",N
+2022-11-07 23:27:59,LilacLight,Col 132 Sector QF-L c9-5,"1163,8125","-49,78125","-744,875",Col 132 Sector QF-L c9-6,"1158,84375","-55,9375","-740,25","9,2",N
+2022-11-07 23:29:11,Gadnok,Synuefe VE-Y c17-20,"147,65625","-100,96875","-295,53125",Synuefe UP-U b36-3,"144,65625","-95,84375","-269,625","26,6",N
+2022-11-07 23:31:10,LilacLight,Col 132 Sector QF-L c9-7,"1156,96875","-56,125","-744,8125",Col 132 Sector QF-L c9-8,"1150,375",-55,"-744,40625","6,7",N
+2022-11-08 00:03:29,LilacLight,Col 132 Sector RF-L c9-10,"1212,71875","-41,6875","-712,3125",Col 132 Sector QF-L c9-5,"1163,8125","-49,78125","-744,875","59,3",N
+2022-11-08 00:27:44,LilacLight,NGC 2232 Sector PD-I b10-0,"762,15625","-146,46875","-896,78125",NGC 2232 Sector IM-L b8-0,"735,90625","-175,875","-944,96875","62,3",N
+2022-11-08 01:29:18,LilacLight,Wregoe LF-B b0,"917,3125","101,78125","-1050,125",Wregoe XM-X b1-0,971,"92,46875","-1005,875","70,2",N
+2022-11-08 01:32:09,LilacLight,Wregoe XM-X b1-0,971,"92,46875","-1005,875",Wregoe AY-V b2-0,"986,65625","103,03125","-998,84375","20,2",N
+2022-11-08 03:48:25,Finnanator252,Col 285 Sector HV-W b2-2,"-31,59375","-45,375","-297,65625",HIP 22460,"-41,3125","-58,96875","-354,78125","59,5",N
+2022-11-08 03:49:38,Mass 1nhibited,California Sector FB-X c1-8,"-342,90625","-208,34375","-932,3125",California Sector JH-V c2-9,"-318,40625","-216,6875","-896,40625","44,3",N
+2022-11-08 03:55:05,Mass 1nhibited,California Sector JH-V c2-9,"-318,40625","-216,6875","-896,40625",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375","8,9",N
+2022-11-08 04:09:18,Mass 1nhibited,HIP 18077,"-303,84375","-236,125","-860,59375",California Sector HR-W d1-27,"-329,625","-219,0625","-887,375","40,9",N
+2022-11-08 04:12:16,Mass 1nhibited,California Sector HR-W d1-27,"-329,625","-219,0625","-887,375",California Sector HR-W d1-28,"-329,53125","-218,5","-893,71875","6,4",N
+2022-11-08 12:53:33,JoDaHammer,Swoiwns BC-B d1-34,"-1243,25","-72,6875","296,9375",Swoiwns AJ-C c2-3,"-1250,4375","-51,65625","310,15625","25,9",N
+2022-11-08 13:11:32,JoDaHammer,Swoiwns AS-E b4-7,"-1245,8125","-53,90625","307,71875",Swoiwns AS-E b4-2,"-1254,96875","-56,96875","311,40625","10,3",Y
+2022-11-08 13:14:36,JoDaHammer,Swoiwns AS-E b4-2,"-1254,96875","-56,96875","311,40625",Swoiwns AS-E b4-7,"-1245,8125","-53,90625","307,71875","10,3",N
+2022-11-08 13:21:13,Amy Thyst,Swoiwns WC-E c1-1,"-1250,71875",-41,"292,40625",Swoiwns AS-E b4-7,"-1245,8125","-53,90625","307,71875","20,6",N
+2022-11-08 17:03:10,HAyden eliback,2 Monocerotis,162,"-76,09375","-223,5625",Synuefe UP-U b36-3,"144,65625","-95,84375","-269,625",53,N
+2022-11-08 19:46:41,Mass 1nhibited,HIP 18390,"-299,0625","-229,25","-876,125",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375","22,2",N
+2022-11-08 19:47:39,LilacLight,Col 132 Sector CF-Y b17-2,"1161,3125","-50,75","-735,3125",Col 132 Sector QF-L c9-8,"1150,375",-55,"-744,40625","14,8",N
+2022-11-08 20:36:51,Amy Thyst,Swoiwns WC-E c1-9,"-1245,0625","-59,84375","290,0625",Swoiwns AS-E b4-7,"-1245,8125","-53,90625","307,71875","18,6",N
+2022-11-08 21:52:52,Wotherspoon,HIP 21852,"-38,53125","-75,3125","-352,40625",HIP 22460,"-41,3125","-58,96875","-354,78125","16,7",Y
+2022-11-08 22:25:01,Mass 1nhibited,California Sector MH-V b2-0,"-317,15625","-251,96875","-956,28125",California Sector QD-S b4-0,"-320,0625","-223,34375","-921,625",45,N
+2022-11-08 22:27:51,SZCZYPH,Pleiades Sector IH-V c2-13,"-84,15625","-137,5",-328,Asterope,"-80,15625","-144,09375","-333,375","9,4",N
+2022-11-08 22:50:42,Wotherspoon,HIP 21852,"-38,53125","-75,3125","-352,40625",HIP 22460,"-41,3125","-58,96875","-354,78125","16,7",Y
+2022-11-08 23:16:22,StarduckTF,Col 285 Sector DJ-R b5-5,"132,21875","-83,78125","-241,03125",Synuefe UP-U b36-3,"144,65625","-95,84375","-269,625","33,4",N
+2022-11-09 00:05:01,Gadnok,Synuefe UP-U b36-1,"141,21875","-104,5625","-282,75",Synuefe UP-U b36-4,"145,1875","-100,59375","-265,8125","17,8",N
+2022-11-09 00:15:15,GrayC,Maia,"-81,78125","-149,4375","-343,375",Merope,"-78,59375","-149,625","-340,53125","4,3",N
+2022-11-09 00:35:58,Mass 1nhibited,California Sector BA-A e6,"-319,8125","-216,75","-913,46875",California Sector NS-T b3-0,"-344,5625","-243,65625","-940,84375","45,7",N
+2022-11-09 00:37:08,Gadnok,Synuefe ZK-W c18-14,145,"-91,5","-257,53125",Synuefe UP-U b36-3,"144,65625","-95,84375","-269,625","12,9",N
+2022-11-09 01:36:44,Sammi Reid,Synuefai EZ-A b33-0,"-263,6875","-183,9375","-361,375",Mel 22 Sector MF-D b13-2,"-243,875","-183,75","-338,21875","30,5",N
+2022-11-09 02:02:08,Sammi Reid,Synuefe UE-Y c17-20,"132,84375","-102,4375","-274,75",Synuefe UP-U b36-3,"144,65625","-95,84375","-269,625","14,5",N
+2022-11-09 02:11:34,Divji Nosi,Pleiades Sector YA-N b7-3,"-85,84375","-139,3125","-281,71875",Celaeno,"-81,09375","-148,3125","-337,09375","56,3",N
+2022-11-09 02:23:13,Divji Nosi,Maia,"-81,78125","-149,4375","-343,375",Asterope,"-80,15625","-144,09375","-333,375","11,5",N
+2022-11-09 03:02:31,GrayC,Maia,"-81,78125","-149,4375","-343,375",Merope,"-78,59375","-149,625","-340,53125","4,3",N
+2022-11-09 04:15:01,wavelengthinversion,Synuefe UD-Z a68-2,"1,71875","-43,65625","-333,6875",Synuefe SA-C b33-3,"-9,03125","-56,625","-351,46875","24,5",
+2022-11-09 05:33:56,Reyedog,Synuefe VG-A b34-4,-39,"-49,9375","-335,9375",HIP 22460,"-41,3125","-58,96875","-354,78125",21,Y
+2022-11-09 08:47:13,msTheo,Pleiades Sector TK-N b7-3,"-105,21875","-91,09375","-283,34375",Pleiades Sector LY-Q b5-2,"-121,59375","-85,78125","-306,34375","28,7",N
+2022-11-09 12:06:14,msTheo,Pleiades Sector PE-P b6-1,"-108,1875","-100,75","-304,1875",Pleiades Sector LY-Q b5-2,"-121,59375","-85,78125","-306,34375","20,2",N
+2022-11-09 20:59:02,Talisaerin,Synuefe YK-W c18-1,"133,125","-92,0625","-251,78125",Synuefe UP-U b36-3,"144,65625","-95,84375","-269,625","21,6",N
+2022-11-09 21:20:34,Mass 1nhibited,California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","45,3",N
+2022-11-09 21:26:48,Mass 1nhibited,California Sector HR-W d1-28,"-329,53125","-218,5","-893,71875",HIP 18390,"-299,0625","-229,25","-876,125","36,8",N
+2022-11-09 22:04:59,Sammi Reid,NGC 2232 Sector TE-G b11-0,"719,84375","-180,53125","-884,84375",NGC 2232 Sector VE-Q c5-6,"699,5625","-181,3125","-893,75","22,2",N
+2022-11-09 22:14:56,badloop1,Pleiades Sector QO-Q b5-3,"-87,65625","-141,125","-315,28125",Asterope,"-80,15625","-144,09375","-333,375","19,8",N
+2022-11-09 22:32:30,Stefanos,Aries Dark Region IM-V c2-10,"-43,28125","-143,75","-234,125",Pleiades Sector MN-T c3-1,"-69,75","-131,625","-290,4375","63,4",N
+2022-11-09 22:38:10,manakin pie-skoffa,Pleiades Sector LN-S b4-1,"-67,90625","-112,21875","-334,21875",Asterope,"-80,15625","-144,09375","-333,375","34,2",N
+2022-11-09 22:43:50,LilacLight,Col 132 Sector LD-R b21-1,"1027,5","-57,65625","-656,375",Col 132 Sector JX-S b20-2,"1065,8125","-56,8125",-681,"45,6",N
+2022-11-09 22:48:11,manakin pie-skoffa,Pleiades Sector IX-S b4-1,"-60,78125","-79,53125","-344,90625",HIP 22460,"-41,3125","-58,96875","-354,78125",30,Y
+2022-11-09 23:51:08,BlakeA,Celaeno,"-81,09375","-148,3125","-337,09375",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","4,6",
+2022-11-09 23:52:06,BlakeA,Celaeno,"-81,09375","-148,3125","-337,09375",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","4,6",
+2022-11-10 01:11:27,Sammi Reid,Mel 22 Sector MF-D b13-2,"-243,875","-183,75","-338,21875",Mel 22 Sector QL-B b14-2,"-226,1875","-182,15625","-320,5","25,1",N
+2022-11-10 01:20:43,Amy Thyst,Swoiwns BM-G b3-6,"-1163,46875","-60,65625","288,5625",HD 195991,"-1181,28125",-55,"287,625","18,7",N
+2022-11-10 03:07:41,ErroCal,California Sector PD-S b4-0,"-339,1875","-220,875","-924,15625",California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875","28,8",N
+2022-11-10 04:41:21,ErroCal,HIP 18390,"-299,0625","-229,25","-876,125",California Sector JH-V c2-9,"-318,40625","-216,6875","-896,40625","30,7",N
+2022-11-10 14:55:09,caRGOrunner1969,Asterope,"-80,15625","-144,09375","-333,375",HR 1183,"-72,875","-145,09375","-336,96875","8,2",N
+2022-11-10 15:27:57,Sammi Reid,Pleiades Sector ZK-W b2-1,"-66,8125","-68,65625","-382,5625",Synuefe YB-A b34-5,"-13,0625","-73,75","-341,5","67,8",Y
+2022-11-10 15:39:43,Sammi Reid,Synuefe DI-Y b34-0,12,"-76,96875",-319,Pleiades Sector FR-U b3-3,"-26,15625","-78,1875","-348,71875","48,4",N
+2022-11-10 16:02:24,BIA RoS,Pleiades Sector QD-S b4-0,"-59,78125","-163,5","-339,71875",Pleiades Sector CQ-Y c2,"-73,3125","-182,78125","-388,4375","54,1",N
+2022-11-10 17:50:37,AllBall,Taurus Dark Region LN-S b4-1,"-54,125","-57,5625","-425,0625",Taurus Dark Region XF-N b7-1,"-49,78125","-48,625","-366,4375","59,5",N
+2022-11-10 18:23:42,AllBall,Taurus Dark Region XF-N b7-1,"-49,78125","-48,625","-366,4375",HIP 22460,"-41,3125","-58,96875","-354,78125","17,7",Y
+2022-11-10 18:54:20,AllBall,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector CG-X c1-12,"-65,0625","-101,03125","-348,53125","48,7",Y
+2022-11-10 20:07:01,badloop1,Pleiades Sector TJ-Q b5-0,"-76,09375","-147,78125","-308,4375",Asterope,"-80,15625","-144,09375","-333,375","25,5",N
+2022-11-10 22:35:44,Pageknight,Pleiades Sector ER-U b3-0,"-60,375","-80,40625","-361,75",Pleiades Sector JX-S b4-2,"-41,03125","-80,78125","-340,8125","28,5",
+2022-11-10 22:47:15,Pageknight,Pleiades Sector GW-W d1-79,"-13,9375","-86,3125","-309,8125",Pleiades Sector JX-S b4-2,"-41,03125","-80,78125","-340,8125","41,5",
+2022-11-10 23:45:45,The Real Wildcard,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Maia,"-81,78125","-149,4375","-343,375",10,N
+2022-11-10 23:49:49,The Real Wildcard,Maia,"-81,78125","-149,4375","-343,375",Pleiades Sector MN-T c3-9,"-84,25","-125,375","-279,09375","68,7",N
+2022-11-11 00:16:57,GrayC,Pleiades Sector HR-W d1-68,"-68,03125","-117,5","-315,15625",HIP 19072,"-54,28125","-121,03125","-323,0625","16,2",N
+2022-11-11 00:55:05,Roxsonix,Col 285 Sector DJ-R b5-2,"119,84375","-83,15625","-239,15625",Col 285 Sector DJ-R b5-5,"132,21875","-83,78125","-241,03125","12,5",N
+2022-11-11 01:05:22,Roxsonix,Synuefe UP-U b36-3,"144,65625","-95,84375","-269,625",Synuefe YK-W c18-1,"133,125","-92,0625","-251,78125","21,6",N
+2022-11-11 01:11:34,Roxsonix,HIP 23018,"-22,34375","-60,8125","-353,75",HIP 22460,"-41,3125","-58,96875","-354,78125","19,1",Y
+2022-11-11 01:24:11,GrayC,Pleiades Sector WF-N b7-2,"-97,78125","-117,1875","-276,71875",Pleiades Sector SZ-O b6-2,"-97,5","-120,6875","-299,125","22,7",N
+2022-11-11 01:31:37,GrayC,Pleiades Sector SZ-O b6-2,"-97,5","-120,6875","-299,125",Pleiades Sector UU-O b6-3,"-88,78125","-135,875","-304,59375","18,3",N
+2022-11-11 01:35:22,GrayC,Pleiades Sector UU-O b6-3,"-88,78125","-135,875","-304,59375",Pleiades Sector KC-V c2-4,"-88,8125","-148,71875","-321,1875",21,N
+2022-11-11 01:37:34,Divji Nosi,HIP 17694,"-72,25","-152,53125","-338,6875",Pleiades Sector IH-U b3-3,"-50,3125","-108,40625","-347,25",50,N
+2022-11-11 01:40:37,LegendaryHobo,HR 1183,"-72,875","-145,09375","-336,96875",Pleiades Sector MI-S b4-0,"-100,25","-139,71875","-343,1875","28,6",N
+2022-11-11 03:27:07,Mass 1nhibited,Siniang,"354,40625","-386,625","-709,21875",Ronemar,"355,75","-400,5","-707,21875","14,1",N
+2022-11-11 03:32:31,Mass 1nhibited,Balchder,"360,0625","-409,5","-703,65625",Evangelis,"353,75","-419,03125",-710,"13,1",N
+2022-11-11 03:43:16,Mass 1nhibited,Suluo,"381,125","-384,375","-725,84375",Lembass,"385,625","-388,21875","-738,09375","13,6",N
+2022-11-11 04:00:16,Divji Nosi,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",Merope,"-78,59375","-149,625","-340,53125","8,5",N
+2022-11-11 05:11:30,Amy Thyst,Swoiwns TT-L b6,"-1078,625","-54,40625","227,625",Swoiwns ZV-C d53,"-1070,53125","-54,6875","254,5","28,1",N
+2022-11-11 05:28:40,TomCatT,Pleiades Sector QO-Q b5-2,"-90,5625","-136,46875","-316,59375",Maia,"-81,78125","-149,4375","-343,375",31,N
+2022-11-11 05:46:20,LilacLight,Synuefe SF-H c13-9,"897,53125","-60,1875","-492,1875",Synuefe FH-S d5-21,"861,40625","-62,71875","-537,21875","57,8",N
+2022-11-11 07:50:26,caRGOrunner1969,HR 1183,"-72,875","-145,09375","-336,96875",Asterope,"-80,15625","-144,09375","-333,375","8,2",N
+2022-11-11 11:37:36,Sammi Reid,Pleiades Sector GW-W d1-73,"-38,625","-82,65625",-321,Pleiades Sector FR-U b3-3,"-26,15625","-78,1875","-348,71875","30,7",Y
+2022-11-11 12:50:47,LCU No Fool Like One,Col 285 Sector DP-Y b1-5,"-38,03125","-48,71875","-320,9375",HIP 22460,"-41,3125","-58,96875","-354,78125","35,5",Y
+2022-11-11 13:10:14,TomCatT,Asterope,"-80,15625","-144,09375","-333,375",Maia,"-81,78125","-149,4375","-343,375","11,5",N
+2022-11-11 13:27:16,LilacLight,Synuefe FH-S d5-21,"861,40625","-62,71875","-537,21875",Synuefe FL-O b26-2,"812,5","-61,59375","-493,0625","65,9",N
+2022-11-11 14:56:56,LilacLight,Wregoe XD-T d3-24,"681,125","75,34375","-676,5",Wregoe ZM-T b17-0,"683,78125","76,96875","-678,6875","3,8",N
+2022-11-11 15:28:22,HaLfY47,Col 285 Sector IP-V a4-0,"-35,84375","-44,96875","-298,78125",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","55,9",N
+2022-11-11 15:30:38,HaLfY47,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",Y
+2022-11-11 16:00:15,Grimscrub,Col 285 Sector BO-R b5-3,"122,71875","-64,4375","-225,8125",Synuefe YK-W c18-1,"133,125","-92,0625","-251,78125","39,3",N
+2022-11-11 17:12:57,Sammi Reid,Synuefe RP-U b36-4,"83,34375","-96,75","-274,78125",Synuefe CC-R b38-1,"138,84375","-99,3125","-236,03125","67,7",N
+2022-11-11 17:15:49,Sammi Reid,Synuefe CC-R b38-1,"138,84375","-99,3125","-236,03125",Synuefe CC-R b38-4,"152,21875","-93,71875","-240,96875","15,3",N
+2022-11-11 17:23:11,SashOk50196,HIP 22460,"-41,3125","-58,96875","-354,78125",HIP 22711,"-35,75","-60,84375","-366,90625","13,5",Y
+2022-11-11 17:27:00,Sammi Reid,Synuefe CC-R b38-4,"152,21875","-93,71875","-240,96875",Synuefe YK-W c18-1,"133,125","-92,0625","-251,78125",22,N
+2022-11-11 17:36:16,Nathan Kerbonaut,Synuefe WK-U b36-2,"145,25","-105,75","-284,1875",Synuefe YK-W c18-1,"133,125","-92,0625","-251,78125","37,2",N
+2022-11-11 17:38:20,MIBE7070,Merope,"-78,59375","-149,625","-340,53125",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125","8,5",N
+2022-11-11 18:07:55,Ashurson,Hyades Sector KW-V b2-2,"66,25","-82,59375","-217,34375",Synuefe YK-W c18-23,"123,375","-97,625","-255,15625","70,1",N
+2022-11-11 18:52:14,AllBall,Delphi,"-63,59375","-147,40625","-319,09375",Pleiades Sector KS-S b4-3,"-62,90625","-91,96875","-339,21875",59,N
+2022-11-11 18:55:34,AllBall,Pleiades Sector KS-S b4-3,"-62,90625","-91,96875","-339,21875",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","37,8",Y
+2022-11-11 19:08:12,MIBE7070,Pleiades Sector JX-S b4-2,"-41,03125","-80,78125","-340,8125",Pleiades Sector EB-X c1-10,"-85,875","-131,9375","-346,375","68,3",Y
+2022-11-11 19:12:01,MIBE7070,Pleiades Sector EB-X c1-10,"-85,875","-131,9375","-346,375",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125","16,7",N
+2022-11-11 19:28:57,Axmil,Pleiades Sector VK-O b6-0,"-161,03125","-183,53125","-303,75",Synuefai SR-V b35-3,"-212,90625","-177,125","-298,0625","52,6",N
+2022-11-11 19:37:54,debugok,Synuefe YK-W c18-2,"133,15625","-97,59375","-257,40625",Synuefe YK-W c18-1,"133,125","-92,0625","-251,78125","7,9",N
+2022-11-11 19:41:35,Grimscrub,Synuefe YK-W c18-2,"133,15625","-97,59375","-257,40625",Synuefe YK-W c18-1,"133,125","-92,0625","-251,78125","7,9",N
+2022-11-11 19:42:51,ndrv,Synuefe UP-U b36-3,"144,65625","-95,84375","-269,625",Synuefe YK-W c18-1,"133,125","-92,0625","-251,78125","21,6",N
+2022-11-11 19:43:20,ENERGYMR,Pleiades Sector IC-U b3-1,"-90,1875","-135,15625","-348,3125",Asterope,"-80,15625","-144,09375","-333,375","20,1",N
+2022-11-11 20:22:40,Helmut Grokenberger,Hyades Sector RX-T b3-5,"84,15625","-88,71875","-202,34375",Col 285 Sector DJ-R b5-2,"119,84375","-83,15625","-239,15625","51,6",N
+2022-11-11 20:45:51,Grimscrub,Synuefe YK-W c18-1,"133,125","-92,0625","-251,78125",Synuefe YK-W c18-2,"133,15625","-97,59375","-257,40625","7,9",Y
+2022-11-11 20:55:06,Requese,Col 285 Sector KQ-W b2-2,"-19,65625","-76,375","-303,5",Pleiades Sector JX-S b4-2,"-41,03125","-80,78125","-340,8125","43,2",N
+2022-11-11 21:21:37,ENERGYMR,Atlas,"-76,71875","-147,34375","-344,4375",Asterope,"-80,15625","-144,09375","-333,375",12,N
+2022-11-11 21:29:16,Kegaira Ohaya,HR 1752,"-15,46875","-17,78125","-287,9375",Synuefe LD-A c17-1,"-20,5625","-61,75","-344,0625","71,5",N
+2022-11-11 21:30:23,ENERGYMR,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector IH-V c2-7,"-78,46875","-144,9375","-324,9375","8,6",N
+2022-11-11 21:48:11,ENERGYMR,HIP 17692,"-74,125","-143,96875","-328,78125",Merope,"-78,59375","-149,625","-340,53125","13,8",N
+2022-11-11 22:38:41,manakin pie-skoffa,Aries Dark Region LD-S b4-2,"-183,65625","-167,03125","-259,46875",Aries Dark Region JI-S b4-0,"-181,03125","-162,96875","-260,09375","4,9",N
+2022-11-11 22:43:18,Ashurson,Pleiades Sector HR-W d1-68,"-68,03125","-117,5","-315,15625",Pleiades Sector JX-S b4-2,"-41,03125","-80,78125","-340,8125","52,3",N
+2022-11-11 22:52:01,KENSHIRO KASUMI,Synuefe YK-W c18-1,"133,125","-92,0625","-251,78125",Synuefe XV-S b37-3,"126,34375","-88,75","-259,875","11,1",Y
+2022-11-11 22:52:31,Mass 1nhibited,NGC 1514 Sector IM-V b2-0,"-223,46875",-235,"-853,34375",HIP 19201,"-260,6875","-216,46875","-888,5","54,4",N
+2022-11-11 23:01:38,Darius Valkorian,Aries Dark Region DB-X c1-2,"-182,78125","-172,84375","-293,375",Pleiades Sector VK-O b6-2,"-150,40625","-181,625","-302,3125","34,7",N
+2022-11-11 23:18:26,Sayaka,Coalsack Sector IX-K b8-11,"451,6875","-24,3125","336,40625",Musca Dark Region KS-T c3-14,"406,0625","-21,125","302,4375",57,N
+2022-11-11 23:19:38,Helmut Grokenberger,Aries Dark Region EW-W d1-37,"-174,25","-168,25","-242,6875",Aries Dark Region HH-V c2-7,"-176,90625","-159,8125","-251,59375","12,6",N
+2022-11-11 23:28:04,Sammi Reid,Aries Dark Region LD-S b4-2,"-183,65625","-167,03125","-259,46875",Aries Dark Region EW-W d1-37,"-174,25","-168,25","-242,6875","19,3",N
+2022-11-11 23:33:08,Sammi Reid,Pleiades Sector PT-Q b5-3,"-72,53125","-118,96875","-317,6875",HIP 22060,"-30,5","-75,34375","-347,90625","67,7",N
+2022-11-12 00:52:35,THARGOSU,HIP 17403,"-93,6875","-158,96875","-367,625",Pleiades Sector UU-O b6-1,"-86,15625","-138,21875","-302,8125","68,5",N
+2022-11-12 01:27:02,GrayC,Pleiades Sector DL-Y d65,"-83,28125","-151,40625","-347,1875",Maia,"-81,78125","-149,4375","-343,375","4,5",N
+2022-11-12 01:59:30,KnightArch,Synuefe CC-R b38-1,"138,84375","-99,3125","-236,03125",Synuefe YK-W c18-1,"133,125","-92,0625","-251,78125","18,3",N
+2022-11-12 02:27:54,GrayC,Pleiades Sector DL-Y d65,"-83,28125","-151,40625","-347,1875",Pleiades Sector HR-W d1-36,"-67,65625","-150,71875","-333,3125","20,9",N
+2022-11-12 02:28:44,LegendaryHobo,HR 1183,"-72,875","-145,09375","-336,96875",Delphi,"-63,59375","-147,40625","-319,09375","20,3",N
+2022-11-12 02:29:10,----whirlwind----,HR 1183,"-72,875","-145,09375","-336,96875",Pleiades Sector LN-S b4-0,"-66,375","-116,75","-336,125","29,1",
+2022-11-12 02:31:48,GrayC,Pleiades Sector HR-W d1-36,"-67,65625","-150,71875","-333,3125",Delphi,"-63,59375","-147,40625","-319,09375","15,2",N
+2022-11-12 02:47:44,jnTracks,Asterope,"-80,15625","-144,09375","-333,375",HIP 17692,"-74,125","-143,96875","-328,78125","7,6",N
+2022-11-12 03:11:27,GrayC,Pleiades Sector DL-Y d65,"-83,28125","-151,40625","-347,1875",Electra,"-86,0625","-159,9375","-361,65625",17,N
+2022-11-12 03:29:03,----whirlwind----,Pleiades Sector JX-S b4-2,"-41,03125","-80,78125","-340,8125",HIP 22460,"-41,3125","-58,96875","-354,78125","25,9",
+2022-11-12 03:43:31,DawnTreader,Col 285 Sector DJ-R b5-5,"132,21875","-83,78125","-241,03125",Synuefe YK-W c18-1,"133,125","-92,0625","-251,78125","13,6",N
+2022-11-12 04:00:03,----whirlwind----,Pleiades Sector KS-S b4-3,"-62,90625","-91,96875","-339,21875",Pleiades Sector ER-U b3-1,"-55,40625","-71,84375","-348,78125","23,5",
+2022-11-12 04:02:24,----whirlwind----,Pleiades Sector ER-U b3-1,"-55,40625","-71,84375","-348,78125",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","15,4",
+2022-11-12 04:15:48,dart frog,Pleiades Sector OY-Q b5-1,"-49,03125","-99,3125","-321,8125",Pleiades Sector JX-S b4-2,"-41,03125","-80,78125","-340,8125","27,7",N
+2022-11-12 10:11:53,Kanasira,Pleiades Sector CW-U b3-1,"-46,53125","-62,9375","-364,5625",HIP 22460,"-41,3125","-58,96875","-354,78125","11,8",Y
+2022-11-12 10:21:51,Kanasira,Pleiades Sector CW-U b3-1,"-46,53125","-62,9375","-364,5625",HIP 22460,"-41,3125","-58,96875","-354,78125","11,8",Y
+2022-11-12 10:50:01,Kanasira,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector CW-U b3-1,"-46,53125","-62,9375","-364,5625","11,8",Y
+2022-11-12 11:08:43,Sirius Sion,Col 285 Sector IL-X c1-16,"29,71875","-76,96875","-296,3125",Synuefe NY-Z c16-1,"-16,21875","-70,4375","-330,21875","57,5",N
+2022-11-12 13:39:31,Gadnok,Synuefe YV-S b37-5,"139,6875",-87,"-255,375",Synuefe YK-W c18-1,"133,125","-92,0625","-251,78125",9,N
+2022-11-12 13:46:07,dart frog,Aries Dark Region RJ-Q b5-2,"-143,65625","-173,28125","-244,90625",Aries Dark Region EW-W d1-37,"-174,25","-168,25","-242,6875","31,1",N
+2022-11-12 14:10:45,Gadnok,Col 285 Sector OR-V c2-20,"126,59375","-91,3125","-227,71875",Synuefe YK-W c18-1,"133,125","-92,0625","-251,78125","24,9",N
+2022-11-12 14:39:08,manakin pie-skoffa,Pleiades Sector MC-V c2-8,"3,46875","-159,59375","-313,84375",Pleiades Sector QD-S b4-2,"-49,03125","-155,03125","-340,1875","58,9",N
+2022-11-12 14:43:59,Sammi Reid,HIP 27061,"150,375","-90,09375","-244,21875",Synuefe YK-W c18-1,"133,125","-92,0625","-251,78125","18,9",N
+2022-11-12 15:42:16,Sammi Reid,Wredguia WN-B c13-5,"-517,53125","34,5","-497,5625",Wredguia VM-B b27-0,"-521,9375","38,375","-483,28125","15,4",N
+2022-11-12 16:18:13,Marko Lomic,Pleiades Sector LS-T c3-6,"-41,03125","-102,4375","-279,375",Pleiades Sector LS-T c3-12,"-53,0625","-102,5625","-287,84375","14,7",N
+2022-11-12 16:22:26,Sammi Reid,Wredguia US-B c13-4,"-522,125","77,21875","-482,71875",Wredguia VM-B b27-2,"-523,1875","51,71875","-471,75","27,8",N
+2022-11-12 17:10:28,DawnTreader,Col 285 Sector OR-V c2-20,"126,59375","-91,3125","-227,71875",Synuefe YK-W c18-1,"133,125","-92,0625","-251,78125","24,9",N
+2022-11-12 17:41:17,Wotherspoon,Synuefe GX-B c16-6,"-37,25","-55,125","-347,15625",HIP 22410,"-49,46875","-55,25","-360,59375","18,2",Y
+2022-11-12 17:49:18,ENERGYMR,HIP 17692,"-74,125","-143,96875","-328,78125",Merope,"-78,59375","-149,625","-340,53125","13,8",N
+2022-11-12 17:58:22,Wotherspoon,Pleiades Sector LN-S b4-2,"-66,75","-107,5","-333,59375",Pleiades Sector OI-S b4-0,"-63,1875","-135,75","-325,21875","29,7",N
+2022-11-12 18:02:12,StarBeaver,Col 285 Sector GL-X c1-5,"-25,28125","-78,90625","-291,09375",Pleiades Sector GW-W d1-73,"-38,625","-82,65625",-321,33,N
+2022-11-12 18:13:06,LilacLight,Synuefe EI-Y b34-2,"30,28125","-76,3125","-305,4375",Pleiades Sector KX-S b4-2,"-21,71875","-75,28125","-342,9375","64,1",N
+2022-11-12 19:48:47,ENERGYMR,Pleiades Sector HR-W d1-79,"-80,625","-146,65625","-343,25",Merope,"-78,59375","-149,625","-340,53125","4,5",N
+2022-11-12 21:13:20,Aelfgar,HIP 21134,"-39,21875","-84,3125","-325,34375",Pleiades Sector JX-S b4-2,"-41,03125","-80,78125","-340,8125",16,Y
+2022-11-12 21:39:06,Fallharbor,Col 285 Sector DP-Y b1-5,"-38,03125","-48,71875","-320,9375",HIP 22460,"-41,3125","-58,96875","-354,78125","35,5",Y
+2022-11-12 22:21:06,Zweistein2,Asterope,"-80,15625","-144,09375","-333,375",Merope,"-78,59375","-149,625","-340,53125","9,2",N
+2022-11-12 22:31:49,Istarias,Merope,"-78,59375","-149,625","-340,53125",Celaeno,"-81,09375","-148,3125","-337,09375","4,4",N
+2022-11-12 22:35:08,Zurdos,Synuefe KD-A c17-10,"-32,375","-58,1875","-332,6875",HIP 22460,"-41,3125","-58,96875","-354,78125","23,8",Y
+2022-11-12 22:45:32,Mass 1nhibited,HIP 18390,"-299,0625","-229,25","-876,125",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375","22,2",N
+2022-11-12 22:51:50,Mass 1nhibited,California Sector HR-W d1-28,"-329,53125","-218,5","-893,71875",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","22,1",N
+2022-11-12 23:01:23,Istarias,Celaeno,"-81,09375","-148,3125","-337,09375",Merope,"-78,59375","-149,625","-340,53125","4,4",N
+2022-11-12 23:03:33,Sammi Reid,Pleiades Sector GW-W d1-73,"-38,625","-82,65625",-321,Pleiades Sector GW-W d1-72,"-22,375","-84,03125","-323,78125","16,5",Y
+2022-11-12 23:57:41,Sammi Reid,Pleiades Sector AH-L b8-0,"-132,875","-128,9375","-259,96875",Aries Dark Region HH-V c2-13,"-180,03125","-163,5625","-231,375","65,1",N
+2022-11-13 01:30:56,ArNeo,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",Pleiades Sector PD-S b4-0,"-80,625","-156,6875","-337,4375","11,5",N
+2022-11-13 01:45:46,Divji Nosi,HIP 17694,"-72,25","-152,53125","-338,6875",Merope,"-78,59375","-149,625","-340,53125","7,2",N
+2022-11-13 01:58:12,ArNeo,Atlas,"-76,71875","-147,34375","-344,4375",Pleiades Sector KC-V c2-9,"-88,71875","-147,8125","-339,0625","13,2",N
+2022-11-13 02:32:28,BlakeA,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125","5,8",
+2022-11-13 03:17:25,LilacLight,Wregoe LF-O b20-2,"678,65625","75,71875","-615,875",Wregoe AK-R d4-25,"651,15625","69,28125","-640,03125","37,2",N
+2022-11-13 04:00:04,GrayC,Celaeno,"-81,09375","-148,3125","-337,09375",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125","6,1",N
+2022-11-13 04:05:12,nerd does elite,Pleiades Sector IH-V c2-17,"-91,78125","-141,0625","-340,375",Pleiades Sector MI-S b4-1,"-103,375","-138,3125","-339,8125","11,9",N
+2022-11-13 11:17:03,CJMVAUCLUSE,Hyades Sector RC-U b3-0,"121,8125","-73,4375","-199,3125",Col 285 Sector OR-V c2-20,"126,59375","-91,3125","-227,71875","33,9",N
+2022-11-13 13:23:54,Cate Nicholls,HIP 19629,"-60,15625","-83,4375","-286,65625",Pleiades Sector GW-W d1-73,"-38,625","-82,65625",-321,"40,5",N
+2022-11-13 13:25:02,Molan Ryke,Pleiades Sector GW-W d1-73,"-38,625","-82,65625",-321,Pleiades Sector ND-R b5-1,"-38,65625","-83,1875","-323,34375","2,4",Y
+2022-11-13 14:32:15,Gray Z7Z,42 n Persei,"-83,5625","-73,40625","-244,34375",Pleiades Sector SZ-O b6-4,"-90,0625","-120,53125","-294,1875","68,9",N
+2022-11-13 15:30:58,StarduckTF,Delphi,"-63,59375","-147,40625","-319,09375",HIP 17692,"-74,125","-143,96875","-328,78125","14,7",N
+2022-11-13 15:32:24,msTheo,Aries Dark Region EW-W d1-37,"-174,25","-168,25","-242,6875",Aries Dark Region NO-Q b5-1,"-175,6875","-164,28125","-237,90625","6,4",Y
+2022-11-13 15:58:31,StarduckTF,Delphi,"-63,59375","-147,40625","-319,09375",HIP 17692,"-74,125","-143,96875","-328,78125","14,7",N
+2022-11-13 16:02:54,manakin pie-skoffa,HIP 17692,"-74,125","-143,96875","-328,78125",Asterope,"-80,15625","-144,09375","-333,375","7,6",N
+2022-11-13 16:24:34,BlakeA,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","5,8",
+2022-11-13 16:56:26,BlakeA,Pleiades Sector PD-S b4-0,"-80,625","-156,6875","-337,4375",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125","11,5",
+2022-11-13 17:55:48,R.Seaton,Col 285 Sector DP-Y b1-5,"-38,03125","-48,71875","-320,9375",HIP 22460,"-41,3125","-58,96875","-354,78125","35,5",Y
+2022-11-13 18:01:17,BlakeA,Pleiades Sector IH-V c2-16,-92,"-143,5","-341,3125",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125","6,8",
+2022-11-13 18:06:59,Stukjes,Aries Dark Region HH-V c2-8,"-165,8125","-164,625","-225,09375",Aries Dark Region EW-W d1-37,"-174,25","-168,25","-242,6875","19,8",N
+2022-11-13 18:43:48,Dominis,California Sector HR-W d1-29,"-343,59375","-214,84375","-889,4375",California Sector JH-V c2-5,"-335,3125","-198,09375","-892,6875",19,N
+2022-11-13 18:51:41,BlakeA,Taygeta,"-88,5","-159,6875","-366,28125",Pleiades Sector DL-Y d69,"-89,84375","-152,0625","-352,40625","15,9",
+2022-11-13 18:57:52,pokegustavo,Synuefe GZ-M d8-80,"-9,875","-77,40625","-313,8125",Synuefe LD-A c17-3,"-23,03125","-62,875","-339,375","32,2",N
+2022-11-13 18:59:35,CHDEN49,Pleiades Sector UY-R b4-0,"-14,46875","-166,375","-331,375",Pleiades Sector IR-W d1-54,"-2,625","-177,84375","-329,53125","16,6",N
+2022-11-13 19:05:45,BlakeA,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",Pleiades Sector IH-V c2-7,"-78,46875","-144,9375","-324,9375","16,6",
+2022-11-13 19:05:57,BlakeA,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",Pleiades Sector IH-V c2-7,"-78,46875","-144,9375","-324,9375","16,6",
+2022-11-13 19:06:12,pokegustavo,Synuefe LD-A c17-3,"-23,03125","-62,875","-339,375",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",25,Y
+2022-11-13 19:18:02,MGFrank70,Aries Dark Region KX-S b4-4,"-39,59375","-101,25","-261,59375",Pleiades Sector MX-U d2-54,"-53,34375","-122,53125","-260,28125","25,4",N
+2022-11-13 19:26:53,BlakeA,Pleiades Sector PD-S b4-0,"-80,625","-156,6875","-337,4375",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125","11,5",
+2022-11-13 19:27:05,BlakeA,Pleiades Sector PD-S b4-0,"-80,625","-156,6875","-337,4375",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125","11,5",
+2022-11-13 19:39:30,CodexNecro81,Aries Dark Region HH-V c2-0,"-149,34375","-170,59375","-242,34375",Aries Dark Region EW-W d1-37,"-174,25","-168,25","-242,6875",25,N
+2022-11-13 20:29:44,Dominis,California Sector VZ-O b6-0,"-304,6875","-181,375","-879,71875",California Sector VZ-O b6-1,"-302,90625","-181,71875","-865,375","14,5",N
+2022-11-13 20:39:14,DawnTreader,Col 285 Sector HV-W b2-3,"-29,21875","-51,9375","-304,25",Synuefe VG-A b34-4,-39,"-49,9375","-335,9375","33,2",N
+2022-11-13 20:44:41,DawnTreader,Synuefe VG-A b34-4,-39,"-49,9375","-335,9375",HIP 22460,"-41,3125","-58,96875","-354,78125",21,Y
+2022-11-13 20:48:32,DawnTreader,HIP 22460,"-41,3125","-58,96875","-354,78125",Synuefe LD-A c17-0,"-22,84375","-64,0625","-339,09375","24,8",Y
+2022-11-13 20:50:28,ArNeo,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125","5,8",N
+2022-11-13 20:59:39,ArNeo,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",Pleiades Sector KC-V c2-4,"-88,8125","-148,71875","-321,1875","18,3",N
+2022-11-13 21:37:21,Ron Wheeler,Synuefe KX-A a68-1,"-63,625","-40,46875","-339,625",HIP 22460,"-41,3125","-58,96875","-354,78125","32,7",Y
+2022-11-13 21:44:24,Amy Thyst,Synuefai SL-G b58-3,"-847,28125","-63,8125","193,46875",Synuefai UL-G b58-1,"-824,28125","-57,8125","178,75",28,N
+2022-11-13 22:28:29,The Blu Fox,Synuefe YK-W c18-1,"133,125","-92,0625","-251,78125",Col 285 Sector FE-R b5-4,"127,28125","-93,78125","-226,78125","25,7",N
+2022-11-13 22:36:07,The Blu Fox,Synuefe BN-Y b34-5,"8,0625","-55,5","-323,71875",Synuefe GX-B c16-6,"-37,25","-55,125","-347,15625",51,N
+2022-11-13 22:54:31,LCU No Fool Like One,Pleiades Sector SE-P b6-0,"-64,71875","-103,96875","-299,28125",Pleiades Sector WF-N b7-2,"-97,78125","-117,1875","-276,71875","42,2",N
+2022-11-13 23:12:30,ArNeo,Merope,"-78,59375","-149,625","-340,53125",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125","8,5",N
+2022-11-13 23:16:09,LilacLight,Synuefe VE-H b30-7,"774,75","-66,15625","-422,15625",Synuefe MS-K b28-0,"748,6875","-67,40625","-457,46875","43,9",N
+2022-11-13 23:38:22,debugok,Col 285 Sector IB-X d1-96,"126,34375","-86,0625","-235,71875",Col 285 Sector OR-V c2-21,"127,71875","-88,15625","-231,5","4,9",N
+2022-11-13 23:46:43,debugok,Col 285 Sector FE-R b5-5,"127,21875","-99,3125","-230,875",Col 285 Sector FE-R b5-0,"130,96875","-100,59375","-237,34375","7,6",N
+2022-11-13 23:59:10,debugok,Col 285 Sector FE-R b5-5,"127,21875","-99,3125","-230,875",Col 285 Sector FE-R b5-0,"130,96875","-100,59375","-237,34375","7,6",N
+2022-11-14 01:49:09,LegendaryHobo,HIP 19615,"-41,71875","-136,78125","-360,03125",HR 1185,"-64,65625","-148,9375","-330,40625","39,4",N
+2022-11-14 03:00:52,GrayC,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","5,8",N
+2022-11-14 03:49:04,GrayC,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125","5,8",N
+2022-11-14 04:51:28,Mass 1nhibited,HIP 18390,"-299,0625","-229,25","-876,125",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375","22,2",N
+2022-11-14 07:41:05,Grimscrub,Aries Dark Region HH-V c2-9,"-166,53125","-165,71875","-231,15625",Aries Dark Region EW-W d1-37,"-174,25","-168,25","-242,6875","14,1",N
+2022-11-14 09:06:45,Sammi Reid,Pleiades Sector GW-W d1-72,"-22,375","-84,03125","-323,78125",Pleiades Sector GW-W d1-73,"-38,625","-82,65625",-321,"16,5",Y
+2022-11-14 10:29:26,T.J. Kirk,Pleiades Sector IH-V c2-5,"-78,71875","-138,03125","-321,78125",Pleiades Sector OI-T c3-6,"-80,65625","-151,625","-292,375","32,5",N
+2022-11-14 11:36:21,LilacLight,NGC 2232 Sector KC-V d2-18,"665,25","-133,3125","-836,8125",NGC 2232 Sector YQ-C b13-1,"661,28125","-176,90625","-834,375","43,8",N
+2022-11-14 13:15:41,MIBE7070,HIP 17694,"-72,25","-152,53125","-338,6875",Celaeno,"-81,09375","-148,3125","-337,09375","9,9",N
+2022-11-14 14:57:41,Gadnok,Synuefe YK-W c18-1,"133,125","-92,0625","-251,78125",Col 285 Sector OR-V c2-20,"126,59375","-91,3125","-227,71875","24,9",N
+2022-11-14 17:57:44,Gadnok,Synuefe YK-W c18-1,"133,125","-92,0625","-251,78125",Col 285 Sector OR-V c2-20,"126,59375","-91,3125","-227,71875","24,9",N
+2022-11-14 18:29:39,CodexNecro81,Aries Dark Region EW-W d1-37,"-174,25","-168,25","-242,6875",Aries Dark Region HH-V c2-9,"-166,53125","-165,71875","-231,15625","14,1",Y
+2022-11-14 19:09:44,Stukjes,Hyades Sector XJ-Z b3,"-26,34375","-73,6875","-255,78125",Pleiades Sector LS-T c3-9,"-33,65625","-81,125","-298,15625","43,6",N
+2022-11-14 21:58:29,Divji Nosi,HIP 16613,"-63,9375","-186,6875","-325,4375",Pleiades Sector GW-W c1-15,"-76,9375","-153,71875","-346,625","41,3",N
+2022-11-14 23:08:37,Marko Lomic,Col 285 Sector IB-X d1-35,"120,90625","-89,09375","-219,0625",Col 285 Sector OR-V c2-20,"126,59375","-91,3125","-227,71875","10,6",N
+2022-11-14 23:51:42,Stefanos,Pleiades Sector IH-V c2-13,"-84,15625","-137,5",-328,Asterope,"-80,15625","-144,09375","-333,375","9,4",N
+2022-11-15 00:04:39,Kretak,Col 285 Sector OR-V c2-20,"126,59375","-91,3125","-227,71875",Col 285 Sector OR-V c2-21,"127,71875","-88,15625","-231,5","5,1",Y
+2022-11-15 01:56:15,Divji Nosi,HIP 16613,"-63,9375","-186,6875","-325,4375",Pleiades Sector SY-R b4-0,"-49,875","-178,6875","-333,28125",18,N
+2022-11-15 02:01:26,Amra Rocannon,Asterope,"-80,15625","-144,09375","-333,375",HIP 17692,"-74,125","-143,96875","-328,78125","7,6",N
+2022-11-15 03:18:28,TomCatT,Maia,"-81,78125","-149,4375","-343,375",Asterope,"-80,15625","-144,09375","-333,375","11,5",N
+2022-11-15 04:42:02,Hootman,Col 285 Sector JK-P b6-3,"118,875","-91,9375","-212,96875",Col 285 Sector OR-V c2-20,"126,59375","-91,3125","-227,71875","16,7",N
+2022-11-15 09:02:49,LittleBigYin,Hyades Sector MH-V c2-19,"134,75","-98,71875","-160,59375",Col 285 Sector IB-X d1-47,"133,875","-94,34375","-206,9375","46,6",N
+2022-11-15 09:26:26,LittleBigYin,Synuefe LD-A c17-3,"-23,03125","-62,875","-339,375",HIP 22460,"-41,3125","-58,96875","-354,78125","24,2",Y
+2022-11-15 13:24:01,Mass 1nhibited,HIP 18390,"-299,0625","-229,25","-876,125",California Sector JH-V c2-12,"-314,34375","-221,71875","-890,34375","22,2",N
+2022-11-15 13:44:10,Sammi Reid,Pleiades Sector LS-T c3-9,"-33,65625","-81,125","-298,15625",Hyades Sector XJ-Z b3,"-26,34375","-73,6875","-255,78125","43,6",N
+2022-11-15 15:26:46,Radiumio,Musca Dark Region LY-Q b5-9,"389,25","-5,59375","263,1875",Musca Dark Region PJ-P b6-1,"432,625","2,53125","288,6875",51,N
+2022-11-15 16:01:58,GCSpitfire,Musca Dark Region EI-Q a6-2,"364,15625","-33,875","223,5625",Musca Dark Region GX-S b4-5,"402,9375","-1,59375","254,21875",59,N
+2022-11-15 16:06:45,PanPiper,Pleiades Sector GW-W d1-73,"-38,625","-82,65625",-321,Pleiades Sector IX-S b4-4,"-53,125","-71,6875","-337,8125","24,8",Y
+2022-11-15 17:11:15,allan mandragoran,Celaeno,"-81,09375","-148,3125","-337,09375",Asterope,"-80,15625","-144,09375","-333,375","5,7",N
+2022-11-15 17:32:55,SashOk50196,Col 285 Sector OR-V c2-20,"126,59375","-91,3125","-227,71875",Col 285 Sector FE-R b5-4,"127,28125","-93,78125","-226,78125","2,7",Y
+2022-11-15 17:44:46,Carsie,Musca Dark Region BQ-Y d37,"375,78125","2,40625","244,625",HIP 62154,"411,375","9,09375","261,46875","39,9",
+2022-11-15 17:52:39,LilacLight,Wregoe IG-F c11-7,"592,78125","69,40625","-580,25",Wregoe WY-G b24-2,"554,71875","62,21875","-536,65625","58,3",N
+2022-11-15 17:53:16,AllBall,HIP 62154,"411,375","9,09375","261,46875",HIP 62270,"413,75","3,46875","264,6875","6,9",N
+2022-11-15 18:21:23,debugok,Col 285 Sector OR-V c2-20,"126,59375","-91,3125","-227,71875",Col 285 Sector FE-R b5-4,"127,28125","-93,78125","-226,78125","2,7",Y
+2022-11-15 18:24:39,Marko S Ramius,Col 285 Sector OR-V c2-20,"126,59375","-91,3125","-227,71875",Col 285 Sector FE-R b5-4,"127,28125","-93,78125","-226,78125","2,7",Y
+2022-11-15 18:29:50,Marko S Ramius,Col 285 Sector FE-R b5-4,"127,28125","-93,78125","-226,78125",Col 285 Sector OR-V c2-20,"126,59375","-91,3125","-227,71875","2,7",N
+2022-11-15 18:57:40,AllBall,Musca Dark Region MO-F a12-2,"436,71875","-42,34375","280,1875",Musca Dark Region MO-F a12-4,"437,25","-44,375","279,3125","2,3",N
+2022-11-15 19:58:10,AllBall,Musca Dark Region HM-V c2-16,"443,90625","-19,34375","278,28125",Musca Dark Region PJ-P b6-1,"432,625","2,53125","288,6875","26,7",N
+2022-11-15 20:51:24,EmulatedPenguin,Pleiades Sector GM-M b7-2,"-62,28125","-189,9375","-278,78125",Pleiades Sector LC-V c2-7,"-61,875","-164,875","-307,5","38,1",N
+2022-11-15 22:22:48,ArNeo,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",Pleiades Sector HR-W d1-30,"-97,46875","-136,90625","-322,34375","22,5",N
+2022-11-15 22:35:34,ArNeo,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",Pleiades Sector HR-W d1-30,"-97,46875","-136,90625","-322,34375","22,5",N
+2022-11-15 22:39:51,ArNeo,Pleiades Sector SZ-O b6-0,"-102,1875","-123,875","-304,40625",Pleiades Sector RZ-O b6-3,"-105,15625","-110,03125","-285,84375","23,3",N
+2022-11-15 23:44:08,Divji Nosi,HIP 15954,"-63,21875","-176,21875","-285,6875",HIP 16613,"-63,9375","-186,6875","-325,4375","41,1",N
+2022-11-16 01:39:35,Divji Nosi,HIP 16568,"-50,46875","-195,0625","-315,15625",HIP 16613,"-63,9375","-186,6875","-325,4375","18,9",N
+2022-11-16 02:35:09,Ashurson,Musca Dark Region PJ-P b6-1,"432,625","2,53125","288,6875",HIP 62138,"450,1875","-15,0625","286,46875",25,N
+2022-11-16 10:51:32,LilacLight,Synuefe VU-Q b24-0,516,"-182,625","-540,09375",Synuefe KI-U b22-1,"469,09375","-175,6875","-571,625","56,9",N
+2022-11-16 12:56:51,AllBall,Musca Dark Region HM-V c2-34,"436,25","-0,28125","287,71875",Musca Dark Region HM-V c2-33,"435,5625","-2,09375","293,59375","6,2",N
+2022-11-16 13:11:07,AllBall,Musca Dark Region DI-G a12-0,"432,65625","0,6875","284,15625",Coalsack Sector VU-O b6-6,"418,65625","24,25","304,5625","34,2",N
+2022-11-16 13:59:45,dart frog,Sterope II,"-81,65625","-147,28125","-340,84375",Delphi,"-63,59375","-147,40625","-319,09375","28,3",N
+2022-11-16 15:30:36,dart frog,HR 1185,"-64,65625","-148,9375","-330,40625",Delphi,"-63,59375","-147,40625","-319,09375","11,5",N
+2022-11-16 16:25:04,caRGOrunner1969,Asterope,"-80,15625","-144,09375","-333,375",HR 1183,"-72,875","-145,09375","-336,96875","8,2",N
+2022-11-16 16:58:40,GCSpitfire,HIP 62154,"411,375","9,09375","261,46875",Musca Dark Region BQ-Y d86,"413,375","2,875","266,4375","8,2",N
+2022-11-16 18:05:43,GCSpitfire,Musca Dark Region LD-R b5-2,"416,71875","3,0625","260,8125",Musca Dark Region LD-R b5-7,"419,28125","4,5",261,"2,9",N
+2022-11-16 19:08:32,EmulatedPenguin,Pleiades Sector LX-U c2-6,"-120,65625","-206,6875","-332,375",Pleiades Sector SE-Q b5-2,"-136,25","-177,8125","-323,5625",34,N
+2022-11-16 19:44:13,BlakeA,HIP 17497,"-79,125","-158,6875","-349,125",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",17,
+2022-11-16 20:13:28,BlakeA,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",Celaeno,"-81,09375","-148,3125","-337,09375","6,1",
+2022-11-16 20:19:40,BlakeA,Celaeno,"-81,09375","-148,3125","-337,09375",Pleiades Sector GW-W c1-13,"-77,375","-156,3125","-352,03125","17,3",
+2022-11-16 20:28:10,BlakeA,Pleiades Sector GW-W c1-13,"-77,375","-156,3125","-352,03125",Merope,"-78,59375","-149,625","-340,53125","13,4",
+2022-11-16 20:43:13,debugok,Col 285 Sector IB-X d1-35,"120,90625","-89,09375","-219,0625",Col 285 Sector OR-V c2-20,"126,59375","-91,3125","-227,71875","10,6",N
+2022-11-16 20:54:13,Cate Nicholls,Aries Dark Region FW-W d1-37,"-130,65625","-134,625","-195,75",Aries Dark Region SU-O b6-0,"-162,25","-163,3125","-221,15625","49,7",N
+2022-11-16 21:55:36,BlakeA,Merope,"-78,59375","-149,625","-340,53125",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125","8,5",
+2022-11-16 22:38:41,Enzix,Hyades Sector UI-S b4-0,"98,28125","-84,5625","-179,65625",Col 285 Sector IB-X d1-35,"120,90625","-89,09375","-219,0625","45,7",N
+2022-11-16 23:09:37,BlakeA,Pleiades Sector KC-V c2-4,"-88,8125","-148,71875","-321,1875",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","14,1",
+2022-11-16 23:24:54,Gray Z7Z,Pleiades Sector MN-T c3-3,-100,"-115,96875","-274,25",Celaeno,"-81,09375","-148,3125","-337,09375","73,2",N
+2022-11-16 23:28:01,Wotherspoon,Pleiades Sector MY-Q b5-3,"-90,21875","-90,0625","-319,09375",HIP 22410,"-49,46875","-55,25","-360,59375","67,8",N
+2022-11-17 02:23:37,Sammi Reid,Wredguia UG-D b26-2,"-462,1875","37,8125","-490,34375",Wredguia BU-Z c13-11,"-502,34375","48,78125","-448,40625","59,1",N
+2022-11-17 02:37:51,Zorya Hopper,HIP 62154,"411,375","9,09375","261,46875",Musca Dark Region CQ-Y d68,"425,4375","1,15625","274,28125","20,6",N
+2022-11-17 04:45:17,HENRY KISSINGER,HIP 25335,"89,8125","-75,34375","-190,1875",Col 285 Sector IB-X d1-35,"120,90625","-89,09375","-219,0625","44,6",N
+2022-11-17 07:40:47,mARKmOSSLEY,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",Y
+2022-11-17 07:45:53,mARKmOSSLEY,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",Y
+2022-11-17 08:22:23,mARKmOSSLEY,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",Y
+2022-11-17 08:58:19,mARKmOSSLEY,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",Y
+2022-11-17 12:13:26,SashOk50196,Col 285 Sector IB-X d1-35,"120,90625","-89,09375","-219,0625",Col 285 Sector JK-P b6-5,"124,8125","-85,875","-215,59375","6,1",Y
+2022-11-17 15:09:55,CP_Luiz,Musca Dark Region RE-P b6-4,"429,6875","-12,84375","285,78125",Musca Dark Region PJ-P b6-1,"432,625","2,53125","288,6875","15,9",N
+2022-11-17 15:41:55,AllBall,HIP 64063,"431,90625","-13,71875",303,Musca Dark Region VK-N b7-8,"433,9375","-14,0625","298,3125","5,1",N
+2022-11-17 19:02:35,matheusgames,Pleiades Sector ND-R b5-4,"-29,84375","-69,0625","-324,75",HIP 22460,"-41,3125","-58,96875","-354,78125","33,7",Y
+2022-11-17 20:24:36,EmulatedPenguin,Pleiades Sector MS-T b3-0,"-100,375","-184,78125","-361,375",HIP 16704,"-118,4375","-170,78125","-387,375","34,6",N
+2022-11-17 21:44:49,EmulatedPenguin,HIP 17583,"-92,09375","-153,78125","-366,21875",Asterope,"-80,15625","-144,09375","-333,375","36,3",N
+2022-11-17 23:28:55,Sammi Reid,Hyades Sector BQ-Y d60,"-1,96875","-74,75","-261,4375",Hyades Sector XJ-Z b3,"-26,34375","-73,6875","-255,78125",25,N
+2022-11-18 00:42:15,Andartu,Synuefe WK-U b36-3,"148,625","-109,71875","-281,40625",Col 285 Sector FE-R b5-1,"120,03125","-97,65625","-237,03125","54,2",N
+2022-11-18 00:56:10,Hrimfaxy,Pleiades Sector DG-X c1-11,"-49,25","-70,4375","-360,8125",HIP 22711,"-35,75","-60,84375","-366,90625","17,6",Y
+2022-11-18 01:19:55,Hrimfaxy,HIP 22711,"-35,75","-60,84375","-366,90625",HIP 22460,"-41,3125","-58,96875","-354,78125","13,5",Y
+2022-11-18 01:24:25,GrayC,HR 1183,"-72,875","-145,09375","-336,96875",Maia,"-81,78125","-149,4375","-343,375","11,8",N
+2022-11-18 01:38:34,Hrimfaxy,HIP 22460,"-41,3125","-58,96875","-354,78125",HIP 22410,"-49,46875","-55,25","-360,59375","10,7",Y
+2022-11-18 02:35:11,Roger Acewell,Col 285 Sector IB-X d1-35,"120,90625","-89,09375","-219,0625",Hyades Sector QC-U b3-2,"113,59375","-82,90625","-200,84375","20,6",Y
+2022-11-18 04:10:19,DawnTreader,Col 285 Sector IB-X d1-35,"120,90625","-89,09375","-219,0625",Col 285 Sector JK-P b6-3,"118,875","-91,9375","-212,96875",7,Y
+2022-11-18 05:13:25,Mass 1nhibited,California Sector BV-Y c7,"-342,3125","-219,28125","-952,71875",California Sector MX-T b3-0,"-312,4375","-211,9375","-932,59375","36,8",N
+2022-11-18 10:48:51,JoDaHammer,Musca Dark Region OY-Q b5-9,"443,6875","-15,46875","264,8125",Musca Dark Region PJ-P b6-1,"432,625","2,53125","288,6875","31,9",N
+2022-11-18 11:03:10,Stukjes,Col 285 Sector OR-V c2-20,"126,59375","-91,3125","-227,71875",Col 285 Sector IB-X d1-35,"120,90625","-89,09375","-219,0625","10,6",N
+2022-11-18 12:02:48,Moonloop,Hyades Sector YJ-Z b2,-21,"-69,625","-251,15625",Hyades Sector XJ-Z b3,"-26,34375","-73,6875","-255,78125","8,2",N
+2022-11-18 12:45:38,JoDaHammer,Pleiades Sector IH-V c2-13,"-84,15625","-137,5",-328,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",15,N
+2022-11-18 15:43:15,ENERGYMR,Pleiades Sector PD-S b4-0,"-80,625","-156,6875","-337,4375",Pleiades Sector MN-T c3-15,"-79,53125","-144,625","-288,65625","50,3",N
+2022-11-18 18:17:52,JoDaHammer,Asterope,"-80,15625","-144,09375","-333,375",Celaeno,"-81,09375","-148,3125","-337,09375","5,7",N
+2022-11-18 18:26:09,Amy Thyst,Synuefai FH-P b53-4,"-705,03125","-54,40625","89,21875",Synuefai ST-G c27-1,"-693,65625","-55,46875","120,0625","32,9",N
+2022-11-18 20:22:07,Iridium Nova,Pleiades Sector CQ-Y d69,"-44,96875","-81,21875","-386,0625",Pleiades Sector FR-U b3-0,"-38,4375",-69,"-359,96875","29,5",Y
+2022-11-18 20:31:50,Iridium Nova,Pleiades Sector FR-U b3-0,"-38,4375",-69,"-359,96875",HIP 22460,"-41,3125","-58,96875","-354,78125","11,7",Y
+2022-11-18 21:07:01,pokegustavo,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",Y
+2022-11-18 21:17:37,pokegustavo,Pleiades Sector FC-T b4-4,"-74,125","-54,78125","-344,15625",HIP 22460,"-41,3125","-58,96875","-354,78125","34,7",Y
+2022-11-18 21:25:31,pokegustavo,Pleiades Sector EC-T b4-0,-98,"-58,8125","-335,28125",HIP 22460,"-41,3125","-58,96875","-354,78125","59,9",N
+2022-11-18 23:08:43,Wotherspoon,Synuefe AN-Y b34-0,-22,"-61,6875","-322,375",HIP 22410,"-49,46875","-55,25","-360,59375","47,5",Y
+2022-11-18 23:16:40,pokegustavo,Musca Dark Region FT-E a13-0,"426,96875","6,5625","287,3125",Musca Dark Region MO-P b6-6,"412,03125","20,875","283,875",21,N
+2022-11-18 23:24:08,pokegustavo,Musca Dark Region FT-E a13-0,"426,96875","6,5625","287,3125",Musca Dark Region MO-P b6-6,"412,03125","20,875","283,875",21,N
+2022-11-18 23:28:34,Dogsbody D,Suluo,"381,125","-384,375","-725,84375",Onoros,"389,71875","-379,9375","-724,15625","9,8",N
+2022-11-18 23:35:41,Dogsbody D,Onoros,"389,71875","-379,9375","-724,15625",Witch Head Sector RY-R b4-0,"365,0625","-413,8125","-711,1875","43,9",N
+2022-11-18 23:37:41,Kretak,Col 285 Sector JK-P b6-3,"118,875","-91,9375","-212,96875",Col 285 Sector IB-X d1-35,"120,90625","-89,09375","-219,0625",7,N
+2022-11-18 23:40:51,pokegustavo,Musca Dark Region MO-P b6-6,"412,03125","20,875","283,875",HIP 62154,"411,375","9,09375","261,46875","25,3",N
+2022-11-18 23:57:52,Kretak,Col 285 Sector JK-P b6-3,"118,875","-91,9375","-212,96875",Col 285 Sector IB-X d1-35,"120,90625","-89,09375","-219,0625",7,N
+2022-11-19 08:18:15,Carsie,Pleiades Sector IH-V c2-18,"-88,34375","-143,78125","-338,84375",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","6,8",
+2022-11-19 15:35:47,Arramall,2 Pi-2 Orionis,"36,875","-81,28125",-206,Hyades Sector FB-X c1-14,-16,"-73,1875","-218,375","54,9",N
+2022-11-19 16:32:08,kotie,Celaeno,"-81,09375","-148,3125","-337,09375",Asterope,"-80,15625","-144,09375","-333,375","5,7",N
+2022-11-19 19:12:50,Ashurson,Omega Carinae,"314,21875","-67,15625","116,8125",Musca Dark Region IR-W d1-88,"474,15625","-25,59375","296,375",244,N
+2022-11-19 19:28:11,JohnnyGTO,Pleiades Sector IR-W c1-1,"-93,90625","-185,375","-359,25",HIP 16753,"-88,3125","-172,9375","-348,84375","17,2",N
+2022-11-19 20:13:45,msTheo,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Pleiades Sector MN-T c3-11,"-93,65625","-121,46875",-288,"53,3",N
+2022-11-19 21:59:22,Sammi Reid,Wredguia LG-W c15-7,"-405,125","37,6875","-381,5625",Wredguia PL-U b30-1,"-432,09375","47,5","-385,65625",29,N
+2022-11-19 22:42:35,PercyNoMercy,HIP 62154,"411,375","9,09375","261,46875",Coalsack Sector IR-W d1-116,"425,5625","0,28125","331,84375","72,3",N
+2022-11-20 00:30:51,msTheo,Asterope,"-80,15625","-144,09375","-333,375",HIP 18244,"-48,625","-131,625","-291,9375","53,5",N
+2022-11-20 00:33:33,blueeagle66,Hyades Sector IW-V b2-2,"19,3125","-72,09375","-224,6875",Hyades Sector FB-X c1-14,-16,"-73,1875","-218,375","35,9",N
+2022-11-20 03:02:45,Gray Z7Z,Pleiades Sector HR-W d1-74,"-87,40625","-150,90625","-344,96875",HIP 17694,"-72,25","-152,53125","-338,6875","16,5",N
+2022-11-20 15:41:46,Ashurson,Musca Dark Region IR-W d1-88,"474,15625","-25,59375","296,375",Coalsack Sector VU-O b6-6,"418,65625","24,25","304,5625",75,N
+2022-11-20 17:26:14,lemla kelib,Col 285 Sector IB-X d1-35,"120,90625","-89,09375","-219,0625",Hyades Sector QC-U b3-4,"111,59375","-83,59375",-196,"25,5",N
+2022-11-20 17:26:30,Black Taurus,Pleiades Sector IX-S b4-0,"-61,25","-84,25","-341,875",HIP 22460,"-41,3125","-58,96875","-354,78125","34,7",Y
+2022-11-20 17:39:31,pokegustavo,HIP 62154,"411,375","9,09375","261,46875",Musca Dark Region PJ-P b6-10,"425,09375","-0,28125","286,9375","30,4",N
+2022-11-20 17:58:17,pokegustavo,Musca Dark Region PJ-P b6-10,"425,09375","-0,28125","286,9375",Musca Dark Region PJ-P b6-6,"419,59375","10,46875",275,17,N
+2022-11-20 18:08:06,Karl berg,Pleiades Sector CB-W b2-1,"-102,0625",-119,"-380,84375",Pleiades Sector AF-Z b2,"-62,84375","-147,03125","-410,375","56,5",N
+2022-11-20 18:34:42,pokegustavo,Musca Dark Region OJ-P b6-8,"414,6875","6,03125","278,6875",HIP 62154,"411,375","9,09375","261,46875","17,8",N
+2022-11-20 19:14:41,lemla kelib,Synuefe FP-W b35-2,"486,21875","-83,71875","-286,9375",Synuefe HP-W b35-3,"517,75","-74,53125","-299,5","35,2",N
+2022-11-20 19:43:26,lemla kelib,Synuefe HP-W b35-3,"517,75","-74,53125","-299,5",Synuefe EF-Y c17-7,"527,90625","-72,875","-296,53125","10,7",N
+2022-11-20 21:29:44,PanPiper,Pleiades Sector GW-W d1-54,"-46,625","-91,6875","-271,03125",Hyades Sector EB-X c1-11,"-26,15625","-72,03125","-220,875","57,6",N
+2022-11-20 21:43:44,Wotherspoon,Col 285 Sector FE-R b5-5,"127,21875","-99,3125","-230,875",Hyades Sector QC-U b3-4,"111,59375","-83,59375",-196,"41,3",N
+2022-11-20 23:17:16,pokegustavo,Musca Dark Region PJ-P b6-1,"432,625","2,53125","288,6875",Musca Dark Region PJ-P b6-10,"425,09375","-0,28125","286,9375","8,2",N
+2022-11-20 23:57:11,pokegustavo,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",Y
+2022-11-21 00:50:57,Kurama Phoenix,Synuefe KV-U b36-7,"495,15625","-82,21875","-273,15625",Synuefe EF-Y c17-7,"527,90625","-72,875","-296,53125","41,3",N
+2022-11-21 05:04:32,msTheo,Pleiades Sector MN-T c3-12,"-70,65625","-119,75","-302,8125",Pleiades Sector PT-Q b5-2,"-74,90625","-119,1875","-313,96875",12,N
+2022-11-21 06:38:11,LilacLight,Wregoe MS-B c13-7,"436,90625","56,3125","-465,34375",Wregoe OY-X b28-2,"464,375","50,28125","-439,875","37,9",N
+2022-11-21 07:30:41,LilacLight,Wregoe MX-L d7-119,"445,5","46,0625","-415,625",Wregoe OY-X b28-2,"464,375","50,28125","-439,875",31,N
+2022-11-21 10:22:50,ClydeSHenry,Pleiades Sector HR-W d1-79,"-80,625","-146,65625","-343,25",Celaeno,"-81,09375","-148,3125","-337,09375","6,4",N
+2022-11-21 15:43:38,Sammi Reid,Hyades Sector RX-T b3-3,"90,53125","-103,3125","-201,8125",Hyades Sector QC-U b3-4,"111,59375","-83,59375",-196,"29,4",N
+2022-11-21 15:44:24,Elfener,Pleiades Sector WE-Q b5-0,"-62,625","-181,9375","-320,34375",HIP 16613,"-63,9375","-186,6875","-325,4375","7,1",N
+2022-11-21 19:44:15,EmulatedPenguin,Pleiades Sector IW-W c1-10,"-23,09375","-184,875","-379,4375",HIP 18730,"-24,125","-186,6875","-369,25","10,4",N
+2022-11-21 21:43:42,MalloryXXX,Pleiades Sector NN-T c3-9,"-43,21875","-130,25","-265,15625",Pleiades Sector IH-V c2-9,"-72,5625","-141,96875","-326,28125","68,8",N
+2022-11-22 00:05:12,GrayC,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",Pleione,-77,"-146,78125","-344,125","10,8",N
+2022-11-22 00:46:43,Vallysa,Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625",Hyades Sector XD-S b4-1,"122,5","-85,34375",-179,31,N
+2022-11-22 01:00:10,Vallysa,Hyades Sector VI-S b4-0,"115,875","-81,25","-181,09375",Hyades Sector QC-U b3-5,"105,875","-81,25","-189,34375",13,N
+2022-11-22 01:14:13,Gadnok,Hyades Sector QC-U b3-5,"105,875","-81,25","-189,34375",Hyades Sector QC-U b3-4,"111,59375","-83,59375",-196,"9,1",N
+2022-11-22 01:23:21,blueeagle66,Hyades Sector LC-U b3-2,"-0,125","-77,15625","-200,6875",Hyades Sector GW-V b2-5,"-13,1875","-75,25","-206,625","14,5",N
+2022-11-22 03:47:44,Eralm,Synuefe SR-L a62-1,"15,59375","-26,25","-400,4375",Synuefe OX-A a68-1,"-17,15625","-37,6875","-341,1875","68,7",N
+2022-11-22 05:22:35,GraphicEqualizer,Hyades Sector GW-V b2-5,"-13,1875","-75,25","-206,625",HIP 20769,"-7,78125","-71,34375","-199,5625","9,7",Y
+2022-11-22 09:50:28,iMetal,HIP 23969,"388,59375","-399,65625","-760,1875",Witch Head Sector JR-W c1-0,"376,46875","-461,8125","-744,0625","65,3",N
+2022-11-22 10:13:52,ClydeSHenry,Celaeno,"-81,09375","-148,3125","-337,09375",Pleiades Sector HR-W d1-79,"-80,625","-146,65625","-343,25","6,4",N
+2022-11-22 11:47:54,TomCatT,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector TZ-O b6-0,"-80,40625","-124,125","-304,125","35,4",N
+2022-11-22 16:40:12,Poperrap,HR 1172,"-75,1875","-152,40625","-346,59375",HIP 17497,"-79,125","-158,6875","-349,125","7,8",N
+2022-11-22 17:08:44,Sammi Reid,Col 285 Sector CG-N b7-0,"-168,3125","-139,03125","-202,90625",Aries Dark Region YV-M b7-2,"-152,8125","-167,90625","-188,875","35,6",N
+2022-11-22 17:26:00,quinnrz,Hyades Sector QC-U b3-4,"111,59375","-83,59375",-196,Hyades Sector QC-U b3-3,"110,875","-82,3125","-198,75","3,1",Y
+2022-11-22 17:33:20,quinnrz,Hyades Sector QC-U b3-4,"111,59375","-83,59375",-196,Hyades Sector QC-U b3-5,"105,875","-81,25","-189,34375","9,1",Y
+2022-11-22 17:47:23,Bommy,Pleione,-77,"-146,78125","-344,125",HIP 17694,"-72,25","-152,53125","-338,6875","9,2",N
+2022-11-22 18:50:22,dart frog,Hyades Sector YO-Q b5-4,"102,59375","-81,09375","-153,65625",Hyades Sector AK-Q b5-3,"106,9375","-88,21875","-152,625","8,4",N
+2022-11-22 19:03:20,dart frog,Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625",HIP 24465,"105,78125","-94,65625","-159,625","12,4",N
+2022-11-22 19:27:27,Vanpourix,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875","8,4",
+2022-11-22 19:53:19,manakin pie-skoffa,Hyades Sector OS-T c3-0,"98,78125","-59,28125","-144,09375",Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625","29,3",N
+2022-11-22 21:11:01,Epaphus,Pentam,"85,375","-47,21875","-129,59375",Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625","49,4",N
+2022-11-22 21:21:17,Epaphus,Hyades Sector QC-U b3-4,"111,59375","-83,59375",-196,Hyades Sector HW-W d1-74,"104,125","-87,15625","-171,5625","25,8",N
+2022-11-22 21:45:18,Pete Justice,Hyades Sector HW-W d1-76,"130,0625","-85,34375","-163,09375",Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625","31,6",N
+2022-11-22 22:50:39,Gosht,Lovaroju,"94,875","-87,84375","-144,71875",Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625","15,3",N
+2022-11-22 23:45:28,Gosht,Hyades Sector QN-T c3-21,"102,125","-97,125","-138,4375",Lovaroju,"94,875","-87,84375","-144,71875","13,3",N
+2022-11-22 23:47:05,dart frog,HIP 25388,"82,4375","-66,34375","-148,34375",Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625","26,6",N
+2022-11-23 02:45:47,GrayC,HR 1172,"-75,1875","-152,40625","-346,59375",Maia,"-81,78125","-149,4375","-343,375","7,9",N
+2022-11-23 03:52:13,Sammi Reid,Hyades Sector MC-U b3-4,"23,78125","-73,21875","-198,125",Hyades Sector GW-V b2-5,"-13,1875","-75,25","-206,625",38,N
+2022-11-23 09:44:32,ClydeSHenry,HIP 23414,"60,125","-78,75","-175,15625",Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625","42,1",N
+2022-11-23 10:19:50,Foperider,Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625",Hyades Sector HW-W d1-74,"104,125","-87,15625","-171,5625","13,8",N
+2022-11-23 11:19:29,Bravestunt,Pleiades Sector SD-T b3-1,"-93,75","-226,1875","-354,53125",Pleiades Sector IR-W c1-0,"-91,96875","-190,53125","-356,875","35,8",N
+2022-11-23 12:31:29,Foperider,Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625",Hyades Sector HW-W d1-74,"104,125","-87,15625","-171,5625","13,8",N
+2022-11-23 15:06:18,psykit,HIP 22460,"-41,3125","-58,96875","-354,78125",Synuefe GX-B c16-5,"-32,9375","-57,5625","-348,15625","10,8",
+2022-11-23 15:12:23,psykit,Synuefe GX-B c16-5,"-32,9375","-57,5625","-348,15625",HIP 22460,"-41,3125","-58,96875","-354,78125","10,8",
+2022-11-23 15:30:41,psykit,HIP 22460,"-41,3125","-58,96875","-354,78125",Synuefe GX-B c16-5,"-32,9375","-57,5625","-348,15625","10,8",
+2022-11-23 15:54:46,Louis Calvert,Pleiades Sector YP-O b6-1,"-55,25","-147,625","-299,375",Delphi,"-63,59375","-147,40625","-319,09375","21,4",N
+2022-11-23 16:17:59,Hurix,Synuefe NY-Z c16-18,"-15,28125","-74,65625","-342,9375",HIP 22711,"-35,75","-60,84375","-366,90625","34,4",Y
+2022-11-23 16:33:56,Andartu,Synuefe LD-A c17-4,"-22,6875","-62,375",-339,HIP 22460,"-41,3125","-58,96875","-354,78125","24,6",Y
+2022-11-23 16:36:31,Andartu,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector CQ-Y d59,"-40,8125","-75,4375","-365,53125","19,7",Y
+2022-11-23 16:46:34,Andartu,Pleiades Sector CQ-Y d59,"-40,8125","-75,4375","-365,53125",Pleiades Sector CW-U b3-2,"-46,6875","-60,0625","-361,1875",17,Y
+2022-11-23 17:33:41,dart frog,Hyades Sector YO-Q b5-2,"111,15625","-80,34375","-157,625",Hyades Sector YO-Q b5-3,"105,28125","-76,09375","-157,1875","7,3",N
+2022-11-23 18:25:46,pokegustavo,Hyades Sector VO-Z b1,"-30,375","-64,25","-252,21875",Hyades Sector GW-V b2-5,"-13,1875","-75,25","-206,625",50,N
+2022-11-23 18:45:37,pokegustavo,Wregoe RL-A b41-0,"400,4375","-20,625","-183,28125",Synuefe PF-L d9-20,"406,5625","-66,75","-203,3125","50,7",N
+2022-11-23 19:03:17,XenosAurion,Pleiades Sector QD-S b4-1,"-57,21875","-149,375","-337,125",Delphi,"-63,59375","-147,40625","-319,09375","19,2",N
+2022-11-23 19:37:01,XenosAurion,Delphi,"-63,59375","-147,40625","-319,09375",HR 1185,"-64,65625","-148,9375","-330,40625","11,5",N
+2022-11-23 19:51:38,pokegustavo,Synuefai OH-P b53-6,"-525,0625","-56,15625","84,9375",Synuefai OH-P b53-7,"-527,0625","-58,8125","87,21875",4,N
+2022-11-23 21:36:47,pokegustavo,Pleiades Sector LD-R b5-3,"-74,78125","-76,40625","-318,15625",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","47,4",N
+2022-11-23 22:02:47,pokegustavo,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",Pleiades Sector DG-X c1-11,"-49,25","-70,4375","-360,8125","13,9",Y
+2022-11-23 22:11:27,pokegustavo,Pleiades Sector DG-X c1-11,"-49,25","-70,4375","-360,8125",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","13,9",Y
+2022-11-23 22:18:37,pokegustavo,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",Pleiades Sector DG-X c1-11,"-49,25","-70,4375","-360,8125","13,9",Y
+2022-11-23 22:37:33,allan mandragoran,Pleiades Sector XF-N b7-3,"-69,3125","-112,9375","-277,59375",Pleiades Sector HR-W d1-60,"-87,40625","-125,21875","-325,75","52,9",N
+2022-11-24 00:21:40,Andartu,Aries Dark Region QT-R c4-9,"-118,46875","-156,1875","-173,625",HIP 10201,"-118,59375","-153,53125","-178,3125","5,4",N
+2022-11-24 00:52:00,dart frog,Hyades Sector YO-Q b5-2,"111,15625","-80,34375","-157,625",Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625","13,2",N
+2022-11-24 01:20:08,Roger Acewell,Mitaimici,"27,9375","-42,09375","-162,28125",Hyades Sector DG-X c1-5,"-4,21875",-64,"-199,875","54,1",N
+2022-11-24 02:02:31,Pageknight,Col 285 Sector HV-W b2-3,"-29,21875","-51,9375","-304,25",HIP 22460,"-41,3125","-58,96875","-354,78125","52,4",N
+2022-11-24 03:13:44,Historia-g,Col 285 Sector EP-Y b1-5,-17,"-51,46875","-307,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","53,7",N
+2022-11-24 07:19:08,Sammi Reid,Hyades Sector TI-S b4-1,"82,46875","-84,71875","-169,0625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","19,7",N
+2022-11-24 07:35:51,mojoxftn1,Trianguli Sector ZJ-Z a2,"78,65625","-43,90625","-122,0625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","59,6",N
+2022-11-24 07:45:52,113mand311,Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","5,4",N
+2022-11-24 07:53:41,113mand311,Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","5,4",N
+2022-11-24 08:53:03,ClydeSHenry,Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","5,4",N
+2022-11-24 09:14:48,00rt,Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","5,4",N
+2022-11-24 09:31:15,LilacLight,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",Hyades Sector HW-W d1-74,"104,125","-87,15625","-171,5625",11,Y
+2022-11-24 10:38:00,GrayC,HR 1172,"-75,1875","-152,40625","-346,59375",Pleiades Sector HR-W d1-36,"-67,65625","-150,71875","-333,3125","15,4",N
+2022-11-24 10:48:41,Poperrap,HIP 27986,"117,34375","-59,125","-163,15625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","27,5",N
+2022-11-24 10:49:40,koishukaze,Hyades Sector QN-T c3-12,"100,65625","-77,21875","-138,90625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","24,4",N
+2022-11-24 11:07:29,GrayC,Merope,"-78,59375","-149,625","-340,53125",Celaeno,"-81,09375","-148,3125","-337,09375","4,4",N
+2022-11-24 12:23:41,Sammi Reid,Aries Dark Region WF-N b7-3,"-106,25","-139,71875","-185,28125",Aries Dark Region QT-R c4-9,"-118,46875","-156,1875","-173,625","23,6",N
+2022-11-24 12:49:19,Solace39,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",Hyades Sector YO-Q b5-3,"105,28125","-76,09375","-157,1875","8,9",Y
+2022-11-24 13:04:00,Red Munchkin,Hyades Sector LH-V c2-20,"91,5625","-88,96875","-157,375",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","13,4",N
+2022-11-24 13:29:50,Hisaki51,Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","5,4",N
+2022-11-24 13:41:32,Hudiny,Hyades Sector AK-Q b5-1,"104,34375","-97,6875","-157,21875",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","17,8",N
+2022-11-24 13:58:58,Sammi Reid,Aries Dark Region QT-R c4-5,"-106,1875","-151,125","-174,03125",Aries Dark Region QT-R c4-12,"-124,15625","-159,71875","-170,90625","20,2",N
+2022-11-24 13:59:42,Draconis andromedae,Hyades Sector DQ-O b6-3,"90,90625","-86,65625","-135,59375",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","29,6",N
+2022-11-24 14:07:51,Slipi,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625","5,4",Y
+2022-11-24 14:18:11,Serraphix,Hyades Sector SD-G a12-2,"99,21875","-44,78125","-146,65625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","39,9",N
+2022-11-24 14:26:06,Hisaki51,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625","5,4",Y
+2022-11-24 14:28:23,Goidbyte,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625","5,4",Y
+2022-11-24 14:36:56,Sammi Reid,Aries Dark Region QT-R c4-12,"-124,15625","-159,71875","-170,90625",Col 285 Sector UT-R c4-11,"-124,0625","-153,65625","-166,65625","7,4",N
+2022-11-24 14:37:22,Louis Calvert,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625","5,4",Y
+2022-11-24 14:37:34,Toqueville,Hyades Sector WT-Q b5-0,"99,46875","-61,125","-164,65625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","20,2",N
+2022-11-24 14:41:56,Hisaki51,Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","5,4",N
+2022-11-24 14:50:05,tokisu,Hyades Sector AA-P b6-0,"100,90625","-64,6875","-143,78125",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","25,3",N
+2022-11-24 15:35:03,THARGOSU,HIP 17403,"-93,6875","-158,96875","-367,625",Pleiades Sector JH-V c2-1,"-50,0625","-126,71875","-326,9375","67,8",N
+2022-11-24 15:43:42,MIBE7070,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",Pleione,-77,"-146,78125","-344,125","10,8",N
+2022-11-24 16:07:08,Historia-g,Hyades Sector XZ-O b6-6,"50,0625","-64,90625","-138,03125",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","58,9",N
+2022-11-24 16:15:09,Anger Ash,Hyades Sector CV-O b6-5,"103,34375","-81,625","-143,3125",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","19,8",N
+2022-11-24 16:30:28,SashOk50196,Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","5,4",N
+2022-11-24 16:36:57,ClydeSHenry,Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","5,4",N
+2022-11-24 16:54:22,Elowen Halcyon,Hyades Sector CV-O b6-5,"103,34375","-81,625","-143,3125",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","19,8",N
+2022-11-24 17:01:03,SashOk50196,Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","5,4",N
+2022-11-24 17:02:07,Zweistein2,Cabrigenses,"77,71875","-56,84375","-158,46875",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","33,9",N
+2022-11-24 17:04:44,SashOk50196,Hyades Sector YO-Q b5-4,"102,59375","-81,09375","-153,65625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","9,4",N
+2022-11-24 17:05:05,Stormknott,No Chang,"66,5625","-44,1875","-120,625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","65,9",N
+2022-11-24 17:11:54,Sammi Reid,HIP 24289,"58,53125","-67,53125","-186,6875",Hyades Sector EB-W b2-4,"-5,78125","-57,71875","-206,34375",68,N
+2022-11-24 17:18:11,g1t,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",Hyades Sector AK-Q b5-3,"106,9375","-88,21875","-152,625","13,8",Y
+2022-11-24 17:32:44,PSB,Hyades Sector MH-V c2-18,"98,5625","-69,6875","-172,625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","15,2",N
+2022-11-24 17:33:35,ndrv,Hyades Sector GW-W d1-87,"77,59375","-74,84375","-136,03125",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","36,2",N
+2022-11-24 18:05:43,PSB,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",HR 1919,"111,28125","-74,28125","-155,5","14,5",Y
+2022-11-24 18:28:40,-|John Wick|-,Senocidi,"78,8125","-77,8125","-139,59375",Hyades Sector LH-V c2-20,"91,5625","-88,96875","-157,375","24,6",N
+2022-11-24 18:34:22,MGFrank70,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",Hyades Sector XO-Q b5-2,"87,59375","-70,3125","-152,6875",20,Y
+2022-11-24 18:34:26,Poperrap,78 Theta-2 Tauri,"1,75","-54,90625",-140,Hyades Sector JH-U b3-6,"-4,5","-63,90625","-190,53125","51,7",N
+2022-11-24 18:51:03,MGFrank70,Hyades Sector XO-Q b5-2,"87,59375","-70,3125","-152,6875",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",20,N
+2022-11-24 18:51:38,DRifter Macraken,Hyades Sector XO-Q b5-2,"87,59375","-70,3125","-152,6875",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",20,
+2022-11-24 19:01:08,Andy Arkwright,Hyades Sector YO-Q b5-6,"111,28125","-66,625","-151,375",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","21,3",Y
+2022-11-24 19:04:06,Gabolander,Hyades Sector YO-Q b5-3,"105,28125","-76,09375","-157,1875",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","8,9",Y
+2022-11-24 19:12:58,Solace39,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",Hyades Sector YO-Q b5-3,"105,28125","-76,09375","-157,1875","8,9",Y
+2022-11-24 19:14:37,Gabolander,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625","5,4",Y
+2022-11-24 19:14:37,HalfFireFortress,Hyades Sector GW-W d1-81,"80,6875","-59,9375","-135,65625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","40,1",Y
+2022-11-24 19:45:54,Carsie,Popongo,"117,6875","-86,5","-125,875",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",41,N
+2022-11-24 20:03:01,Seamus Donohue,Hyades Sector YO-Q b5-4,"102,59375","-81,09375","-153,65625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","9,4",Y
+2022-11-24 20:26:10,Seamus Donohue,Lovaroju,"94,875","-87,84375","-144,71875",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","20,3",Y
+2022-11-24 20:34:02,Solace39,Hyades Sector YO-Q b5-3,"105,28125","-76,09375","-157,1875",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","8,9",Y
+2022-11-24 20:38:57,Jaden Capek,Ixbalan,"87,8125","-81,34375","-136,25",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","29,7",Y
+2022-11-24 20:41:02,psolem,Hyades Sector LH-V c2-16,"91,78125","-71,46875","-168,75",Hyades Sector MH-V c2-18,"98,5625","-69,6875","-172,625",8,Y
+2022-11-24 20:46:11,Flaw less,Woyeru,"77,5625","-64,09375","-160,15625",Hyades Sector LH-V c2-16,"91,78125","-71,46875","-168,75","18,2",Y
+2022-11-24 20:47:53,Solace39,Hyades Sector YO-Q b5-3,"105,28125","-76,09375","-157,1875",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","8,9",Y
+2022-11-24 20:49:04,Jaden Capek,Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625",Hyades Sector AK-Q b5-3,"106,9375","-88,21875","-152,625","11,2",Y
+2022-11-24 21:01:38,Cate Nicholls,Hyades Sector QN-T c3-13,"99,0625","-68,53125","-139,4375",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","26,8",N
+2022-11-24 21:14:21,BlakeA,Celaeno,"-81,09375","-148,3125","-337,09375",Maia,"-81,78125","-149,4375","-343,375","6,4",
+2022-11-24 21:42:37,psykit,HIP 22460,"-41,3125","-58,96875","-354,78125",Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125","5,2",
+2022-11-24 21:45:38,psykit,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",
+2022-11-24 21:48:40,psykit,Pleiades Sector BL-X c1-7,"-44,75","-61,125","-351,53125",HIP 22460,"-41,3125","-58,96875","-354,78125","5,2",
+2022-11-24 22:04:14,Carsie,Hyades Sector UI-S b4-6,"97,21875","-68,90625","-179,1875",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","20,7",Y
+2022-11-24 22:06:13,Gabolander,Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","5,4",Y
+2022-11-24 22:06:19,Molan Ryke,Hyades Sector GW-V b2-5,"-13,1875","-75,25","-206,625",HIP 20769,"-7,78125","-71,34375","-199,5625","9,7",N
+2022-11-24 22:16:16,BramleyHouse,Nihal,"98,96875","-72,125","-103,53125",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","60,1",N
+2022-11-24 22:23:36,Carsie,HIP 27305,"112,53125","-64,78125","-157,15625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","20,9",Y
+2022-11-24 22:48:21,Historia-g,Hyades Sector XZ-O b6-6,"50,0625","-64,90625","-138,03125",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","58,9",N
+2022-11-24 22:54:54,Enki Amarok,Hyades Sector QN-T c3-12,"100,65625","-77,21875","-138,90625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","24,4",Y
+2022-11-24 22:55:21,Historia-g,Hyades Sector QC-U b3-4,"111,59375","-83,59375",-196,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","34,8",Y
+2022-11-24 23:08:06,Wotherspoon,Lovaroju,"94,875","-87,84375","-144,71875",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","20,3",Y
+2022-11-24 23:25:20,Solace39,Hyades Sector YO-Q b5-3,"105,28125","-76,09375","-157,1875",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","8,9",Y
+2022-11-24 23:35:21,lAMOTHE,Maia,"-81,78125","-149,4375","-343,375",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",10,N
+2022-11-24 23:35:35,brmsa,Hyades Sector GW-W d1-87,"77,59375","-74,84375","-136,03125",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","36,2",Y
+2022-11-25 00:15:24,Epaphus,Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","5,4",Y
+2022-11-25 00:18:08,Firehawk894,Hyades Sector YO-Q b5-3,"105,28125","-76,09375","-157,1875",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","8,9",Y
+2022-11-25 00:18:57,BlakeA,Maia,"-81,78125","-149,4375","-343,375",Pleiades Sector IH-V c2-7,"-78,46875","-144,9375","-324,9375","19,3",
+2022-11-25 00:27:55,BlakeA,Pleiades Sector IH-V c2-7,"-78,46875","-144,9375","-324,9375",Maia,"-81,78125","-149,4375","-343,375","19,3",
+2022-11-25 00:28:07,BlakeA,Pleiades Sector IH-V c2-7,"-78,46875","-144,9375","-324,9375",Maia,"-81,78125","-149,4375","-343,375","19,3",
+2022-11-25 00:54:20,Orzorzo,Hyades Sector QN-T c3-12,"100,65625","-77,21875","-138,90625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","24,4",Y
+2022-11-25 01:36:56,GrayC,HR 1172,"-75,1875","-152,40625","-346,59375",Pleiades Sector IH-V c2-6,"-77,625","-142,21875","-325,25","23,8",N
+2022-11-25 02:29:31,LilacLight,Synuefe IO-O d7-79,"299,0625","-171,1875",-370,Synuefe VX-A b33-2,"315,46875","-172,15625","-357,375","20,7",N
+2022-11-25 03:27:00,Historia-g,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",Hyades Sector YO-Q b5-4,"102,59375","-81,09375","-153,65625","9,4",Y
+2022-11-25 03:36:43,Historia-g,Hyades Sector YO-Q b5-4,"102,59375","-81,09375","-153,65625",HR 1919,"111,28125","-74,28125","-155,5","11,2",Y
+2022-11-25 03:42:01,Synngularity,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",Hyades Sector HW-W d1-73,"107,09375","-71,53125","-160,34375","11,8",Y
+2022-11-25 04:28:51,pokegustavo,Hyades Sector YO-Q b5-3,"105,28125","-76,09375","-157,1875",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","8,9",Y
+2022-11-25 04:32:50,Molan Ryke,Hyades Sector DG-X c1-5,"-4,21875",-64,"-199,875",HIP 20769,"-7,78125","-71,34375","-199,5625","8,2",N
+2022-11-25 04:41:26,Gadnok,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625","5,4",Y
+2022-11-25 04:42:40,Historia-g,HIP 27305,"112,53125","-64,78125","-157,15625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","20,9",Y
+2022-11-25 04:53:00,Gadnok,Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625",Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375","5,4",Y
+2022-11-25 06:24:49,Sammi Reid,Hyades Sector EG-N b7-0,"103,71875","-62,0625","-122,25",Hyades Sector YO-Q b5-4,"102,59375","-81,09375","-153,65625","36,7",Y
+2022-11-25 08:29:03,LilacLight,Wregoe QD-K d8-18,"443,125","39,96875","-327,8125",Wregoe ZF-W c15-4,"397,3125","41,15625","-367,21875","60,4",N
+2022-11-25 15:14:40,kotie,Celaeno,"-81,09375","-148,3125","-337,09375",Delphi,"-63,59375","-147,40625","-319,09375","25,1",N
+2022-11-25 16:22:58,Sammi Reid,HIP 18609,"-15,96875","-85,65625","-173,90625",HIP 19082,"-20,28125","-81,75","-189,0625","16,2",N
+2022-11-25 17:04:01,LilacLight,HIP 32697,"359,4375","-95,28125","-214,25",Synuefe PF-L d9-16,"375,125","-75,6875","-190,15625","34,8",N
+2022-11-25 17:23:59,Eralm,Hyades Sector BV-O b6-2,"88,875","-71,375","-131,90625",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"10,5",Y
+2022-11-25 19:16:58,FULGEN,Swahku,"91,25","-74,90625","-126,5625",Hyades Sector BV-O b6-2,"88,875","-71,375","-131,90625","6,8",Y
+2022-11-25 21:21:21,Polaras,Aries Dark Region YU-O b6-0,"-31,9375","-147,0625","-224,625",HIP 15954,"-63,21875","-176,21875","-285,6875","74,5",N
+2022-11-25 21:55:03,Last winter,Musca Dark Region IM-V c2-24,"458,25","-8,8125","287,09375",Musca Dark Region ZG-J a10-4,"408,71875","-26,03125","258,125","59,9",N
+2022-11-25 22:19:36,Last winter,Musca Dark Region SV-K a9-4,"362,25","-36,46875","248,53125",Musca Dark Region EN-H a11-3,"415,53125","-26,625","274,25",60,N
+2022-11-25 22:22:17,Last winter,Musca Dark Region EN-H a11-3,"415,53125","-26,625","274,25",Musca Dark Region IM-V c2-24,"458,25","-8,8125","287,09375",48,N
+2022-11-25 22:45:46,felinesaffron32,Hyades Sector PD-J b9-2,"53,1875","-106,25","-79,15625",Trianguli Sector CA-A c22,"75,90625","-71,84375","-114,28125","54,2",N
+2022-11-25 23:02:36,DøømHåmstër,Lovaroju,"94,875","-87,84375","-144,71875",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"26,9",Y
+2022-11-25 23:51:47,Darwin,HIP 18909,"-24,5625","-107,3125","-237,75",Pleiades Sector ZF-N b7-3,"-40,53125","-109,84375","-268,875","35,1",N
+2022-11-26 01:06:18,LilacLight,Synuefe TL-J d10-24,"364,71875","-54,34375","-181,78125",Synuefe XZ-L b41-4,"363,96875",-75,"-182,15625","20,7",N
+2022-11-26 02:54:15,Reyedog,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",Swahku,"91,25","-74,90625","-126,5625","38,2",Y
+2022-11-26 05:57:20,Kurochi,HIP 23022,"-30,375","-51,1875","-338,15625",HIP 22460,"-41,3125","-58,96875","-354,78125","21,4",Y
+2022-11-26 06:01:24,Kurochi,HIP 22460,"-41,3125","-58,96875","-354,78125",HIP 21852,"-38,53125","-75,3125","-352,40625","16,7",Y
+2022-11-26 08:23:47,Sammi Reid,Wredguia XD-K d8-45,"-298,34375","38,1875","-332,78125",Wredguia WD-K d8-44,"-330,375","43,6875","-296,3125","48,8",N
+2022-11-26 09:42:09,MattW,Pleiades Sector AB-N b7-2,"-60,53125","-142,03125","-270,875",HIP 17692,"-74,125","-143,96875","-328,78125","59,5",N
+2022-11-26 09:45:25,MattW,HIP 17692,"-74,125","-143,96875","-328,78125",Asterope,"-80,15625","-144,09375","-333,375","7,6",N
+2022-11-26 11:52:12,LilacLight,Synuefe IO-O d7-79,"299,0625","-171,1875",-370,Synuefe MU-M d8-78,"293,1875","-168,125","-330,84375","39,7",N
+2022-11-26 15:32:39,ENERGYMR,Pleiades Sector IH-V c2-16,-92,"-143,5","-341,3125",Asterope,"-80,15625","-144,09375","-333,375","14,3",N
+2022-11-26 19:06:24,ENERGYMR,Pleiades Sector GW-W c1-15,"-76,9375","-153,71875","-346,625",HR 1172,"-75,1875","-152,40625","-346,59375","2,2",N
+2022-11-26 19:20:02,Michael Vohr,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",Hyades Sector PN-T c3-7,"87,40625","-85,4375","-142,3125",25,Y
+2022-11-26 20:30:32,Deathcow,California Sector IW-W c1-6,"-299,5","-226,5625","-943,34375",California Sector BA-A e6,"-319,8125","-216,75","-913,46875","37,4",N
+2022-11-26 21:01:50,Metcy,Col 285 Sector IV-W b2-4,"-15,1875","-62,4375","-289,84375",Synuefe GX-B c16-6,"-37,25","-55,125","-347,15625","61,8",N
+2022-11-26 21:37:11,Malcotch,Hyades Sector DQ-O b6-3,"90,90625","-86,65625","-135,59375",Swahku,"91,25","-74,90625","-126,5625","14,8",Y
+2022-11-27 00:05:01,Sven Sapphire,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",Ixbalan,"87,8125","-81,34375","-136,25","29,7",Y
+2022-11-27 03:27:35,LegendaryHobo,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",Hyades Sector BV-O b6-2,"88,875","-71,375","-131,90625","34,7",Y
+2022-11-27 04:33:41,DøømHåmstër,Lovaroju,"94,875","-87,84375","-144,71875",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"26,9",Y
+2022-11-27 04:55:56,msTheo,Merope,"-78,59375","-149,625","-340,53125",Maia,"-81,78125","-149,4375","-343,375","4,3",N
+2022-11-27 06:30:07,msTheo,Pleiades Sector HR-W d1-79,"-80,625","-146,65625","-343,25",Pleiades Sector VU-O b6-3,"-71,78125","-141,375","-294,53125","49,8",N
+2022-11-27 10:11:46,ArkannFR49,Aries Dark Region CH-L b8-2,"-105,75","-162,78125","-172,53125",Aries Dark Region QT-R c4-10,"-116,84375","-157,4375","-169,90625","12,6",N
+2022-11-27 14:38:22,Kira Nakatani,Nareg,"71,625","-51,875","-114,28125",Swahku,"91,25","-74,90625","-126,5625","32,7",N
+2022-11-27 18:21:59,Epaphus,Swahku,"91,25","-74,90625","-126,5625",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"5,4",Y
+2022-11-27 18:29:36,Historia-g,HR 1737,"82,5625","-70,78125","-117,21875",Swahku,"91,25","-74,90625","-126,5625","13,4",N
+2022-11-27 18:47:18,Historia-g,HR 1737,"82,5625","-70,78125","-117,21875",Swahku,"91,25","-74,90625","-126,5625","13,4",N
+2022-11-27 18:59:57,Historia-g,Celaeno,"-81,09375","-148,3125","-337,09375",Maia,"-81,78125","-149,4375","-343,375","6,4",N
+2022-11-27 19:02:53,Historia-g,Maia,"-81,78125","-149,4375","-343,375",Merope,"-78,59375","-149,625","-340,53125","4,3",N
+2022-11-27 19:09:34,Historia-g,Merope,"-78,59375","-149,625","-340,53125",Maia,"-81,78125","-149,4375","-343,375","4,3",N
+2022-11-27 19:24:31,Senoreggwardo,HIP 26688,"101,6875","-64,5625","-107,90625",Hyades Sector GB-N b7-1,"98,84375","-77,15625","-118,46875","16,7",N
+2022-11-27 19:35:17,Anger Ash,Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875",Asterope,"-80,15625","-144,09375","-333,375","4,4",N
+2022-11-27 19:54:42,DøømHåmstër,HR 1737,"82,5625","-70,78125","-117,21875",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"8,6",N
+2022-11-27 19:57:28,Seven,Hyades Sector HW-W d1-74,"104,125","-87,15625","-171,5625",Swahku,"91,25","-74,90625","-126,5625","48,4",Y
+2022-11-27 20:06:32,uf,Hyades Sector QN-T c3-11,"96,15625","-82,90625","-142,125",Swahku,"91,25","-74,90625","-126,5625","18,2",Y
+2022-11-27 20:34:19,BlakeA,Pleiades Sector IH-V c2-7,"-78,46875","-144,9375","-324,9375",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125","16,6",
+2022-11-27 20:34:31,BlakeA,Pleiades Sector IH-V c2-7,"-78,46875","-144,9375","-324,9375",Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125","16,6",
+2022-11-27 21:17:19,BlakeA,Pleiades Sector KC-V c2-11,"-86,59375","-147,03125","-339,28125",HIP 16753,"-88,3125","-172,9375","-348,84375","27,7",
+2022-11-27 21:50:48,Reyedog,Ixbalan,"87,8125","-81,34375","-136,25",HR 1737,"82,5625","-70,78125","-117,21875","22,4",Y
+2022-11-27 22:24:32,Wotherspoon,Hupang,"94,84375","-77,625","-116,03125",Swahku,"91,25","-74,90625","-126,5625","11,5",Y
+2022-11-27 22:49:46,Wotherspoon,Matshiru,"94,75","-69,34375","-104,0625",Hupang,"94,84375","-77,625","-116,03125","14,6",N
+2022-11-27 22:53:59,Wotherspoon,Hupang,"94,84375","-77,625","-116,03125",Swahku,"91,25","-74,90625","-126,5625","11,5",Y
+2022-11-27 22:55:20,Moraes,Hyades Sector YO-Q b5-4,"102,59375","-81,09375","-153,65625",Swahku,"91,25","-74,90625","-126,5625",30,Y
+2022-11-27 23:00:08,Ashurson,Awara,"67,21875","-72,65625","-127,34375",Hyades Sector BV-O b6-3,"89,4375","-71,875","-138,96875","25,1",Y
+2022-11-27 23:05:44,Wotherspoon,Hupang,"94,84375","-77,625","-116,03125",Swahku,"91,25","-74,90625","-126,5625","11,5",Y
+2022-11-27 23:08:43,XenosAurion,HR 1185,"-64,65625","-148,9375","-330,40625",Pleiades Sector IH-V c2-7,"-78,46875","-144,9375","-324,9375","15,4",N
+2022-11-27 23:25:09,Firehawk894,Hyades Sector BV-O b6-3,"89,4375","-71,875","-138,96875",Swahku,"91,25","-74,90625","-126,5625","12,9",Y
+2022-11-27 23:55:04,Firehawk894,Hyades Sector AV-O b6-2,"66,25","-84,8125","-139,6875",Swahku,"91,25","-74,90625","-126,5625","29,9",Y
+2022-11-28 00:13:01,Firehawk894,Hyades Sector KH-L b8-0,"98,75","-79,28125","-102,1875",Swahku,"91,25","-74,90625","-126,5625","25,9",N
+2022-11-28 02:22:27,GrayC,Celaeno,"-81,09375","-148,3125","-337,09375",Maia,"-81,78125","-149,4375","-343,375","6,4",N
+2022-11-28 03:05:00,GrayC,Celaeno,"-81,09375","-148,3125","-337,09375",Pleiades Sector HR-W d1-41,"-83,96875","-146,15625","-334,21875","4,6",N
+2022-11-28 05:28:57,SAWAMURA_ERIRI,Hyades Sector BV-O b6-2,"88,875","-71,375","-131,90625",Swahku,"91,25","-74,90625","-126,5625","6,8",Y
+2022-11-28 06:02:49,SAWAMURA_ERIRI,Hyades Sector BV-O b6-2,"88,875","-71,375","-131,90625",Swahku,"91,25","-74,90625","-126,5625","6,8",Y
+2022-11-28 06:12:02,SAWAMURA_ERIRI,Swahku,"91,25","-74,90625","-126,5625",Hyades Sector BV-O b6-2,"88,875","-71,375","-131,90625","6,8",Y
+2022-11-28 06:19:19,Reyedog,Njorog,"92,5625","-61,875","-127,40625",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"14,7",Y
+2022-11-28 06:20:38,Raghav102,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",Swahku,"91,25","-74,90625","-126,5625","38,2",Y
+2022-11-28 06:36:01,SAWAMURA_ERIRI,Swahku,"91,25","-74,90625","-126,5625",Hyades Sector BV-O b6-2,"88,875","-71,375","-131,90625","6,8",Y
+2022-11-28 06:39:28,SAWAMURA_ERIRI,Swahku,"91,25","-74,90625","-126,5625",Hyades Sector BV-O b6-2,"88,875","-71,375","-131,90625","6,8",Y
+2022-11-28 06:49:58,SAWAMURA_ERIRI,Hyades Sector BV-O b6-2,"88,875","-71,375","-131,90625",Swahku,"91,25","-74,90625","-126,5625","6,8",Y
+2022-11-28 06:55:44,SAWAMURA_ERIRI,Swahku,"91,25","-74,90625","-126,5625",Hyades Sector BV-O b6-2,"88,875","-71,375","-131,90625","6,8",Y
+2022-11-28 07:01:59,SAWAMURA_ERIRI,Hyades Sector BV-O b6-2,"88,875","-71,375","-131,90625",Swahku,"91,25","-74,90625","-126,5625","6,8",Y
+2022-11-28 10:10:46,Radiumio,Swahku,"91,25","-74,90625","-126,5625",Hyades Sector BV-O b6-2,"88,875","-71,375","-131,90625","6,8",Y
+2022-11-28 10:13:19,Radiumio,Hyades Sector BV-O b6-2,"88,875","-71,375","-131,90625",Swahku,"91,25","-74,90625","-126,5625","6,8",Y
+2022-11-28 10:54:54,Danuwoa,Pleiades Sector DL-Y d65,"-83,28125","-151,40625","-347,1875",Pleiades Sector SO-Q b5-1,"-46,96875","-135,90625","-311,0625","53,5",N
+2022-11-28 10:56:51,Reyedog,Gandje,"79,78125","-66,875","-131,0625",HR 1737,"82,5625","-70,78125","-117,21875","14,7",Y
+2022-11-28 11:02:50,Reyedog,HR 1737,"82,5625","-70,78125","-117,21875",Swahku,"91,25","-74,90625","-126,5625","13,4",N
+2022-11-28 12:54:42,Radiumio,HIP 25654,"94,78125","-70,46875","-128,5",Swahku,"91,25","-74,90625","-126,5625",6,Y
+2022-11-28 13:57:10,felinesaffron32,HR 1737,"82,5625","-70,78125","-117,21875",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"8,6",N
+2022-11-28 14:00:26,Poperrap,Trianguli Sector GW-V a2-1,"73,90625","-40,90625","-97,6875",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"44,3",N
+2022-11-28 14:07:57,CJMVAUCLUSE,Dirone,"77,25","-44,75","-99,375",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"39,3",N
+2022-11-28 14:08:08,Williamb,Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"39,7",Y
+2022-11-28 14:14:00,Henoch,HR 1604,"66,3125","-69,0625","-90,875",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"38,6",N
+2022-11-28 14:22:12,CJMVAUCLUSE,Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,Swahku,"91,25","-74,90625","-126,5625","5,4",Y
+2022-11-28 14:38:29,Racius,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"43,3",Y
+2022-11-28 15:42:42,Aquanid,Arakak,"41,4375","-40,8125","-104,75",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"60,6",N
+2022-11-28 15:48:31,Foperider,Swahku,"91,25","-74,90625","-126,5625",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"5,4",Y
+2022-11-28 16:08:18,Omnicognate,Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,Swahku,"91,25","-74,90625","-126,5625","5,4",Y
+2022-11-28 16:33:46,Andartu,Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,Swahku,"91,25","-74,90625","-126,5625","5,4",Y
+2022-11-28 16:34:55,Fayr,Ahauduwonai,"93,1875","-84,53125","-74,96875",Swahku,"91,25","-74,90625","-126,5625","52,5",N
+2022-11-28 17:07:37,Molan Ryke,Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,Swahku,"91,25","-74,90625","-126,5625","5,4",Y
+2022-11-28 17:27:10,Omnicognate,Swahku,"91,25","-74,90625","-126,5625",HR 1737,"82,5625","-70,78125","-117,21875","13,4",Y
+2022-11-28 17:34:21,jnTracks,Asterope,"-80,15625","-144,09375","-333,375",HIP 17692,"-74,125","-143,96875","-328,78125","7,6",N
+2022-11-28 17:41:44,MIBE7070,42 Xi Eridani,54,"-116,03125","-165,34375",Swahku,"91,25","-74,90625","-126,5625","67,7",N
+2022-11-28 17:54:33,CP_Luiz,Trianguli Sector QI-S a4-2,"88,28125","-41,21875","-79,59375",Wangakwan,"100,34375","-65,46875","-136,125","62,7",N
+2022-11-28 17:57:03,Radiumio,HR 1737,"82,5625","-70,78125","-117,21875",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"8,6",N
+2022-11-28 18:05:44,Ashurson,Swahku,"91,25","-74,90625","-126,5625",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"5,4",Y
+2022-11-28 18:58:05,Epaphus,Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,Swahku,"91,25","-74,90625","-126,5625","5,4",Y
+2022-11-28 19:01:27,jarp01,HR 1737,"82,5625","-70,78125","-117,21875",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"8,6",N
+2022-11-28 19:06:04,Max Encoder,HR 1737,"82,5625","-70,78125","-117,21875",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"8,6",N
+2022-11-28 19:17:13,ClydeSHenry,HR 1737,"82,5625","-70,78125","-117,21875",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"8,6",N
+2022-11-28 19:24:31,msTheo,HR 1737,"82,5625","-70,78125","-117,21875",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"8,6",N
+2022-11-28 19:27:30,Bravestunt,HIP 25840,"73,9375","-54,125","-112,78125",Swahku,"91,25","-74,90625","-126,5625","30,4",N
+2022-11-28 19:35:52,Ecthelion,Hyades Sector FB-N b7-2,"92,46875","-82,4375","-108,21875",Hupang,"94,84375","-77,625","-116,03125","9,5",N
+2022-11-28 19:41:21,dart frog,Synuefe ND-A c17-8,"60,34375","-63,8125","-316,375",Synuefe TA-C b33-3,"-1,59375","-53,71875",-349,"70,7",N
+2022-11-28 19:43:25,JoDaHammer,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",Swahku,"91,25","-74,90625","-126,5625","38,2",Y
+2022-11-28 20:10:14,Ashurson,Awara,"67,21875","-72,65625","-127,34375",Swahku,"91,25","-74,90625","-126,5625","24,1",Y
+2022-11-28 20:25:12,Epaphus,HR 1737,"82,5625","-70,78125","-117,21875",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"8,6",N
+2022-11-28 20:31:06,dart frog,Pleiades Sector HM-U b3-3,"-35,40625","-97,53125",-358,HIP 22711,"-35,75","-60,84375","-366,90625","37,8",Y
+2022-11-28 20:40:18,Logan Aigaion,Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,Swahku,"91,25","-74,90625","-126,5625","5,4",Y
+2022-11-28 20:50:03,Pete Justice,Trianguli Sector BA-A d84,"65,625","-68,59375","-107,40625",HR 1737,"82,5625","-70,78125","-117,21875","19,7",N
+2022-11-28 20:51:30,Radiumio,HR 1737,"82,5625","-70,78125","-117,21875",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"8,6",N
+2022-11-28 21:06:40,Logan Aigaion,Swahku,"91,25","-74,90625","-126,5625",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"5,4",Y
+2022-11-28 21:18:34,Dan Steele,Hyades Sector GW-M b7-5,"66,625","-94,375","-116,03125",HIP 23816,"87,875","-82,75","-112,59375","24,5",N
+2022-11-28 21:30:49,Logan Aigaion,Swahku,"91,25","-74,90625","-126,5625",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"5,4",Y
+2022-11-28 21:32:37,Sammi Reid,Col 285 Sector WL-S b5-3,"-300,6875","45,8125","-230,375",Wredguia ZC-I b37-1,"-279,9375","45,5","-254,59375","31,9",N
+2022-11-28 21:42:10,Sammi Reid,Arietis Sector DA-Z b3,"-14,09375","-65,71875","-185,15625",Hyades Sector NN-S b4-1,"-0,4375","-61,4375","-180,5","15,1",N
+2022-11-28 21:42:31,wizardkitty,Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,Swahku,"91,25","-74,90625","-126,5625","5,4",Y
+2022-11-28 21:47:08,Sammi Reid,Arietis Sector KW-W b1-1,"-44,03125","-109,96875","-178,71875",Aries Dark Region RT-R c4-13,"-98,34375","-149,15625","-166,1875","68,1",N
+2022-11-28 21:49:19,Epaphus,Trianguli Sector AQ-X a1-1,"48,96875","-37,5","-114,90625",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"54,8",N
+2022-11-28 21:51:11,wizardkitty,Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,Swahku,"91,25","-74,90625","-126,5625","5,4",Y
+2022-11-28 21:54:04,wizardkitty,Hyades Sector FB-N b7-4,"90,9375","-78,78125","-113,25",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"9,9",N
+2022-11-28 22:03:28,PanPiper,Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,Swahku,"91,25","-74,90625","-126,5625","5,4",Y
+2022-11-28 22:11:16,PanPiper,Swahku,"91,25","-74,90625","-126,5625",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"5,4",Y
+2022-11-28 22:23:34,PanPiper,Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,Swahku,"91,25","-74,90625","-126,5625","5,4",Y
+2022-11-28 22:39:46,Amra Rocannon,Hyades Sector YO-Q b5-1,"100,90625","-81,21875","-162,9375",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"43,3",Y
+2022-11-28 22:47:25,SZCZYPH,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector IH-V c2-13,"-84,15625","-137,5",-328,"9,4",N
+2022-11-28 22:52:21,Historia-g,HR 1737,"82,5625","-70,78125","-117,21875",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"8,6",N
+2022-11-28 22:53:31,-|John Wick|-,Swahku,"91,25","-74,90625","-126,5625",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"5,4",Y
+2022-11-28 22:59:36,uf,Swahku,"91,25","-74,90625","-126,5625",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"5,4",Y
+2022-11-28 23:04:34,Chase Hutchins,Trianguli Sector EQ-Y b0,"78,15625","-61,96875","-120,78125",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"16,6",N
+2022-11-28 23:06:21,Moraes,HIP 23128,"61,5625","-70,375","-112,6875",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"28,8",N
+2022-11-28 23:13:09,PanPiper,Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,Swahku,"91,25","-74,90625","-126,5625","5,4",Y
+2022-11-28 23:25:14,SZCZYPH,Asterope,"-80,15625","-144,09375","-333,375",Pleiades Sector IH-V c2-13,"-84,15625","-137,5",-328,"9,4",N
+2022-11-28 23:29:06,wizardkitty,Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,Swahku,"91,25","-74,90625","-126,5625","5,4",Y
+2022-11-28 23:32:18,wizardkitty,Swahku,"91,25","-74,90625","-126,5625",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"5,4",Y
+2022-11-29 00:30:58,-|John Wick|-,Pleiades Sector DL-Y d67,"-80,25","-156,9375","-351,96875",Pleiades Sector GW-W c1-6,"-82,3125",-148,"-358,375","11,2",N
+2022-11-29 00:40:34,Beetlejude,Hyades Sector IM-L b8-5,"104,375","-64,5","-104,625",Hupang,"94,84375","-77,625","-116,03125","19,8",N
+2022-11-29 01:03:45,Iridium Nova,HR 1737,"82,5625","-70,78125","-117,21875",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"8,6",N
+2022-11-29 01:41:38,GrayC,HR 1172,"-75,1875","-152,40625","-346,59375",Maia,"-81,78125","-149,4375","-343,375","7,9",N
+2022-11-29 02:13:31,GrayC,Pleiades Sector GW-W c1-13,"-77,375","-156,3125","-352,03125",Maia,"-81,78125","-149,4375","-343,375","11,9",N
+2022-11-29 02:30:25,wrod288,Hyades Sector YO-Q b5-0,"98,6875","-84,4375","-159,15625",Hyades Sector QN-T c3-12,"100,65625","-77,21875","-138,90625","21,6",Y
+2022-11-29 02:31:51,wizardkitty,Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,Swahku,"91,25","-74,90625","-126,5625","5,4",Y
+2022-11-29 02:35:49,ze ruela,Pleiades Sector IX-S b4-1,"-60,78125","-79,53125","-344,90625",Pleiades Sector ER-U b3-1,"-55,40625","-71,84375","-348,78125","10,1",N
+2022-11-29 02:45:00,GrayC,Maia,"-81,78125","-149,4375","-343,375",Asterope,"-80,15625","-144,09375","-333,375","11,5",N
+2022-11-29 03:23:24,Tumama,Swahku,"91,25","-74,90625","-126,5625",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"5,4",Y
+2022-11-29 03:52:59,Lhorndra,Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,HR 1737,"82,5625","-70,78125","-117,21875","8,6",Y
+2022-11-29 03:54:04,boobtron,HIP 17692,"-74,125","-143,96875","-328,78125",Pleiades Sector IH-V c2-11,"-89,5625","-143,125","-317,21875","19,3",N
+2022-11-29 04:19:42,wizardkitty,Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,Swahku,"91,25","-74,90625","-126,5625","5,4",Y
+2022-11-29 04:46:10,Thorned Rose,Trianguli Sector GG-Y c18,"72,1875","-66,625","-103,3125",HR 1737,"82,5625","-70,78125","-117,21875","17,8",N
+2022-11-29 04:49:34,Mass 1nhibited,Trianguli Sector DQ-Y b4,"61,125","-58,84375","-109,8125",HR 1737,"82,5625","-70,78125","-117,21875","25,6",N
+2022-11-29 05:14:57,Red Munchkin,BD-11 1025,"52,875","-58,3125","-89,875",HR 1737,"82,5625","-70,78125","-117,21875","42,2",N
+2022-11-29 05:18:53,Red Munchkin,HR 1737,"82,5625","-70,78125","-117,21875",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"8,6",N
+2022-11-29 06:04:59,Williamb,Swahku,"91,25","-74,90625","-126,5625",Hyades Sector FB-N b7-6,"88,4375","-74,90625",-122,"5,4",Y
+2022-11-29 06:29:20,Lhorndra,Hyades Sector FB-N b7-4,"90,9375","-78,78125","-113,25",Swahku,"91,25","-74,90625","-126,5625","13,9",N
\ No newline at end of file
diff --git a/data/json_stations.json b/data/json_stations.json
new file mode 100644
index 0000000..07d9d6a
--- /dev/null
+++ b/data/json_stations.json
@@ -0,0 +1,143131 @@
+[
+ {
+ "name": "1 G. Caeli",
+ "pos_x": 80.90625,
+ "pos_y": -83.53125,
+ "pos_z": -30.8125,
+ "stations": "Smoot Gateway,Kandel Arsenal,Giacobini Base"
+ },
+ {
+ "name": "1 Geminorum",
+ "pos_x": 19.78125,
+ "pos_y": 3.5625,
+ "pos_z": -153.8125,
+ "stations": "Collins Settlement,Zholobov Orbital,Seddon Silo"
+ },
+ {
+ "name": "1 Hydrae",
+ "pos_x": 60.90625,
+ "pos_y": 28.53125,
+ "pos_z": -54.90625,
+ "stations": "Chern Barracks,Hieb Orbital,Afanasyev Port,Voss Hub,Whitney Station,Feustel Port,Kepler's Inheritance,Hutton Port,Arber Terminal,Hornby Dock,Yurchikhin Port,Greenleaf's Progress,Weitz's Folly,Littlewood Reach"
+ },
+ {
+ "name": "1 i Centauri",
+ "pos_x": 38.46875,
+ "pos_y": 29.78125,
+ "pos_z": 40.46875,
+ "stations": "Ampere Dock,Armstrong Ring"
+ },
+ {
+ "name": "1 Kappa Cygni",
+ "pos_x": -117.75,
+ "pos_y": 37.78125,
+ "pos_z": 11.1875,
+ "stations": "Wohler Port,Kirtley's Inheritance,Hauck Enterprise,Kinsey Ring"
+ },
+ {
+ "name": "10 Arietis",
+ "pos_x": -79.09375,
+ "pos_y": -88.21875,
+ "pos_z": -105.875,
+ "stations": "Chadwick Station,Morgan Terminal,Hoyle's Folly,Archimedes Base"
+ },
+ {
+ "name": "10 Canum Venaticorum",
+ "pos_x": -9.375,
+ "pos_y": 55.4375,
+ "pos_z": -7,
+ "stations": "He Port,Collins Port,Litke Port,Alvares Bastion,Godwin Installation,Rice Prospect"
+ },
+ {
+ "name": "10 Delta Coronae Borealis",
+ "pos_x": -73.0625,
+ "pos_y": 130.03125,
+ "pos_z": 81.6875,
+ "stations": "Ramaswamy Port"
+ },
+ {
+ "name": "10 G. Canis Majoris",
+ "pos_x": 96.5625,
+ "pos_y": -38.28125,
+ "pos_z": -80.3125,
+ "stations": "Vesalius Port,Rushd Enterprise,Babbage Gateway,Precourt Enterprise,Gohar City,Herreshoff Arsenal,Dupuy de Lome Plant,McAuley's Progress"
+ },
+ {
+ "name": "10 Hydrae",
+ "pos_x": 123.46875,
+ "pos_y": 99.78125,
+ "pos_z": -138.3125,
+ "stations": "Hahn Terminal,Silverberg Survey"
+ },
+ {
+ "name": "10 Iota Cygni",
+ "pos_x": -116.375,
+ "pos_y": 32.03125,
+ "pos_z": 12.625,
+ "stations": "Parise Hub,Euler Ring,Onnes Hub,Eisenstein Plant,Pauli's Folly"
+ },
+ {
+ "name": "10 Kappa Pegasi",
+ "pos_x": -102.40625,
+ "pos_y": -39.75,
+ "pos_z": 19.9375,
+ "stations": "Hubble Gateway,So-yeon Dock,Bondar Hub,Nicollier Terminal,Needham Dock,Herzfeld Dock,Morin Dock,Fife Vista,Saberhagen Base,Stein Port"
+ },
+ {
+ "name": "10 Rho-3 Eridani",
+ "pos_x": 10.96875,
+ "pos_y": -107.375,
+ "pos_z": -82.0625,
+ "stations": "Brandenstein Vision"
+ },
+ {
+ "name": "10 Serpentis",
+ "pos_x": -10.03125,
+ "pos_y": 90.125,
+ "pos_z": 92.59375,
+ "stations": "Chaviano Freeport"
+ },
+ {
+ "name": "10 Tauri",
+ "pos_x": 3.25,
+ "pos_y": -29.9375,
+ "pos_z": -34.1875,
+ "stations": "Clairaut City,McAuley Penal colony,Gaultier de Varennes Enterprise,Bella Laboratory"
+ },
+ {
+ "name": "10 Theta Piscium",
+ "pos_x": -93.59375,
+ "pos_y": -115.375,
+ "pos_z": 0.15625,
+ "stations": "Hovell Mines,Stasheff Horizons,Williamson Mines,Piccard Prospect,McArthur Keep"
+ },
+ {
+ "name": "101 Tauri",
+ "pos_x": 12.0625,
+ "pos_y": -35.375,
+ "pos_z": -127.78125,
+ "stations": "Schmitt Orbital,Baturin City,Frimout Port,Eisele City,Hammond Ring,Linenger Station,Ramon Station"
+ },
+ {
+ "name": "102 Iota Tauri",
+ "pos_x": 3.3125,
+ "pos_y": -34.34375,
+ "pos_z": -169.28125,
+ "stations": "Waldrop Prospect"
+ },
+ {
+ "name": "108 Herculis",
+ "pos_x": -146.34375,
+ "pos_y": 59.1875,
+ "pos_z": 93,
+ "stations": "Cartmill Observatory,Thornycroft Enterprise,Anderson Colony"
+ },
+ {
+ "name": "109 Herculis",
+ "pos_x": -87.96875,
+ "pos_y": 31.09375,
+ "pos_z": 73.78125,
+ "stations": "Bennett Colony,So-yeon Mines"
+ },
+ {
+ "name": "109 Piscium",
+ "pos_x": -51.40625,
+ "pos_y": -69.1875,
+ "pos_z": -62.15625,
+ "stations": "Ising Dock,Jemison Installation"
+ },
+ {
+ "name": "109 Virginis",
+ "pos_x": 6.0625,
+ "pos_y": 106.09375,
+ "pos_z": 82.40625,
+ "stations": "Melvill Gateway"
+ },
+ {
+ "name": "11 Aquilae",
+ "pos_x": -113.84375,
+ "pos_y": 11.09375,
+ "pos_z": 108.90625,
+ "stations": "Markov's Claim,Nielsen Hub,Efremov Camp,Emshwiller Landing"
+ },
+ {
+ "name": "11 Cephei",
+ "pos_x": -167.3125,
+ "pos_y": 43.96875,
+ "pos_z": -57.875,
+ "stations": "Cook Gateway,Bisson Landing"
+ },
+ {
+ "name": "11 Virginis",
+ "pos_x": 56.6875,
+ "pos_y": 131.75,
+ "pos_z": 8,
+ "stations": "Illy Orbital,Thornton Port,Cseszneky Enterprise,Beckman Hub,Wheeler Terminal"
+ },
+ {
+ "name": "110 Herculis",
+ "pos_x": -48,
+ "pos_y": 10.875,
+ "pos_z": 38.75,
+ "stations": "Stirling Vision,Jahn Hangar,Kinsey Arena"
+ },
+ {
+ "name": "111 Tauri",
+ "pos_x": 6.0625,
+ "pos_y": -7.90625,
+ "pos_z": -45.875,
+ "stations": "Gillekens Settlement,Samokutyayev Orbital,Vernadsky Penitentiary"
+ },
+ {
+ "name": "114 G. Aquilae",
+ "pos_x": -97.0625,
+ "pos_y": -28.28125,
+ "pos_z": 79.15625,
+ "stations": "Margulies Prospect,Morey Terminal,Wilcutt Port,Mendeleev Port,Jones Stop,Hirase Gateway,Savery Enterprise,Boole Penal colony"
+ },
+ {
+ "name": "12 Andromedae",
+ "pos_x": -123.46875,
+ "pos_y": -49.625,
+ "pos_z": -32.09375,
+ "stations": "Patterson Hangar,Cauchy Orbital"
+ },
+ {
+ "name": "12 Aquarii",
+ "pos_x": -57.0625,
+ "pos_y": 57.03125,
+ "pos_z": 68.8125,
+ "stations": "Sturgeon Platform,Doctorow Settlement"
+ },
+ {
+ "name": "12 d Bootis",
+ "pos_x": -19.5,
+ "pos_y": 115.8125,
+ "pos_z": 33.1875,
+ "stations": "Nicholson Terminal,Julian Hub,Nagata Terminal,Asher Orbital,Boole's Inheritance,Williamson Enterprise,Shaikh Terminal,Williams Port,Reed Barracks,Nelder Landing"
+ },
+ {
+ "name": "12 G. Canis Minoris",
+ "pos_x": 85.90625,
+ "pos_y": 30.4375,
+ "pos_z": -148.0625,
+ "stations": "Fancher Enterprise,Raleigh Terminal,Gaspar de Lemos Horizons,Neville Vision,King Base"
+ },
+ {
+ "name": "12 Gamma-1 Delphini",
+ "pos_x": -104.5625,
+ "pos_y": -36.03125,
+ "pos_z": 55.59375,
+ "stations": "Walheim Installation,Al-Battani Orbital,Arrhenius Beacon"
+ },
+ {
+ "name": "12 Lambda Coronae Borealis",
+ "pos_x": -76.15625,
+ "pos_y": 103.4375,
+ "pos_z": 43.3125,
+ "stations": "Tucker City,Doctorow Station,Cenker Penal colony,Mitchell Landing"
+ },
+ {
+ "name": "12 Persei",
+ "pos_x": -42.84375,
+ "pos_y": -23.6875,
+ "pos_z": -61.90625,
+ "stations": "Kovalevsky Orbital,Wundt Terminal"
+ },
+ {
+ "name": "12 Sextantis",
+ "pos_x": 118.46875,
+ "pos_y": 133.1875,
+ "pos_z": -79.5,
+ "stations": "Fowler Port,Whymper Stop"
+ },
+ {
+ "name": "127 G. Canis Majoris",
+ "pos_x": 107.875,
+ "pos_y": -9.625,
+ "pos_z": -84.34375,
+ "stations": "Baturin Prospect,Selous Enterprise,Andree's Progress"
+ },
+ {
+ "name": "13 Lambda Crateris",
+ "pos_x": 107.40625,
+ "pos_y": 88.71875,
+ "pos_z": 12.125,
+ "stations": "Morukov Dock,Phillips Hub,Furrer Hub,Fernao do Po Holdings"
+ },
+ {
+ "name": "13 Leonis Minoris",
+ "pos_x": 19.5625,
+ "pos_y": 132.75,
+ "pos_z": -110.4375,
+ "stations": "Andrews Point"
+ },
+ {
+ "name": "13 Orionis",
+ "pos_x": 18.03125,
+ "pos_y": -27,
+ "pos_z": -84.21875,
+ "stations": "Underwood Port,Leiber Prospect,Berezin's Progress,Scott Port,Collins Vision"
+ },
+ {
+ "name": "13 Sextantis",
+ "pos_x": 110.8125,
+ "pos_y": 126.53125,
+ "pos_z": -71.375,
+ "stations": "Balmer Escape"
+ },
+ {
+ "name": "13 Trianguli",
+ "pos_x": -48.75,
+ "pos_y": -47.9375,
+ "pos_z": -76.3125,
+ "stations": "Bartoe Hub,Mendeleev Base,Kerwin Hub,Lee Survey"
+ },
+ {
+ "name": "132 G. Aquarii",
+ "pos_x": -87.03125,
+ "pos_y": -96.40625,
+ "pos_z": 43.40625,
+ "stations": "Hawkes Depot,Chretien Ring,Grabe Gateway"
+ },
+ {
+ "name": "133 G. Canis Major",
+ "pos_x": 136.5,
+ "pos_y": -24.6875,
+ "pos_z": -69.375,
+ "stations": "Dorsett Horizons,Maxwell Terminal,McCarthy Vision"
+ },
+ {
+ "name": "14 Eridani",
+ "pos_x": 15.21875,
+ "pos_y": -86.3125,
+ "pos_z": -68.90625,
+ "stations": "Lamarck Vision,Meucci Station,Leinster Hub,Sarich Stop"
+ },
+ {
+ "name": "14 Geminorum",
+ "pos_x": 12.28125,
+ "pos_y": 5.0625,
+ "pos_z": -57.25,
+ "stations": "Clarke Keep,Gantt Station,Ericsson Port,Artsebarsky Dock"
+ },
+ {
+ "name": "14 Herculis",
+ "pos_x": -36.71875,
+ "pos_y": 41.6875,
+ "pos_z": 14.125,
+ "stations": "Chilton Platform"
+ },
+ {
+ "name": "14 i Orionis",
+ "pos_x": 41.4375,
+ "pos_y": -59.46875,
+ "pos_z": -179.625,
+ "stations": "Veblen Station,Rawn Works,Burroughs Survey"
+ },
+ {
+ "name": "14 Psi Cancri",
+ "pos_x": 35.25,
+ "pos_y": 64.3125,
+ "pos_z": -113.21875,
+ "stations": "Nagel Hub,Nakaya Dock,Potter Terminal,Bushnell Station,Yamazaki Hub,Hale City,Land Enterprise,Xiaoguan Port"
+ },
+ {
+ "name": "14 Tau Ursae Majoris",
+ "pos_x": -46.59375,
+ "pos_y": 81.03125,
+ "pos_z": -84.96875,
+ "stations": "Darnielle Enterprise,Cook Platform,Robinson Laboratory"
+ },
+ {
+ "name": "14 Vulpeculae",
+ "pos_x": -140.46875,
+ "pos_y": -10.53125,
+ "pos_z": 76.28125,
+ "stations": "Gurragchaa Terminal,Fuchs Base"
+ },
+ {
+ "name": "15 Delphini",
+ "pos_x": -80.96875,
+ "pos_y": -33.5,
+ "pos_z": 47.90625,
+ "stations": "Johnson Vision"
+ },
+ {
+ "name": "15 f Leonis",
+ "pos_x": 32,
+ "pos_y": 121.59375,
+ "pos_z": -98.75,
+ "stations": "Hume Vision,Anthony Vision,Gallun Vision,Siodmak Holdings"
+ },
+ {
+ "name": "15 G. Aqr",
+ "pos_x": -72.40625,
+ "pos_y": -77.875,
+ "pos_z": 97.125,
+ "stations": "Laval Prospect"
+ },
+ {
+ "name": "15 Gamma Comae Berenices",
+ "pos_x": 5.3125,
+ "pos_y": 166.625,
+ "pos_z": -13.4375,
+ "stations": "Schmitz Laboratory"
+ },
+ {
+ "name": "15 Lambda Aurigae",
+ "pos_x": -8.5625,
+ "pos_y": 1.5,
+ "pos_z": -40.28125,
+ "stations": "Wiley Station,MacLean City,Appel Enterprise,Helms Orbital,Barnes Reach,Tognini Orbital,Filter's Pride,Benz Ring,Jacquard Station,Jones Relay,Macquorn Rankine City,Ray Ring"
+ },
+ {
+ "name": "15 Pegasi",
+ "pos_x": -83.625,
+ "pos_y": -30.1875,
+ "pos_z": 10.84375,
+ "stations": "Usachov Beacon,Mastracchio's Claim"
+ },
+ {
+ "name": "15 Psi Scorpii",
+ "pos_x": -6.96875,
+ "pos_y": 73.53125,
+ "pos_z": 136.9375,
+ "stations": "Maclaurin Depot,Carter Survey,Thuot Landing"
+ },
+ {
+ "name": "15 Ursae Majoris",
+ "pos_x": -15.90625,
+ "pos_y": 63.59375,
+ "pos_z": -67.375,
+ "stations": "Salam Terminal,Bamford Horizons"
+ },
+ {
+ "name": "157 G. Aquarii",
+ "pos_x": -44.53125,
+ "pos_y": -64.6875,
+ "pos_z": 26.5625,
+ "stations": "Dunbar Hub,Titov Port,Scheerbart's Progress,Lucid City"
+ },
+ {
+ "name": "16 c Ursae Majoris",
+ "pos_x": -21.375,
+ "pos_y": 41.84375,
+ "pos_z": -43.21875,
+ "stations": "Daimler Station,Platt Barracks,Nachtigal Settlement"
+ },
+ {
+ "name": "16 Cephei",
+ "pos_x": -108,
+ "pos_y": 30.03125,
+ "pos_z": -42.25,
+ "stations": "Song Settlement"
+ },
+ {
+ "name": "16 Cygni",
+ "pos_x": -66.5625,
+ "pos_y": 15.53125,
+ "pos_z": 7.5,
+ "stations": "Vonnegut Lab,Borisenko Dock,Siemens Orbital,Hoffman Terminal,Northrop Port,Kaleri Market,Payette Market,Holdstock Arsenal"
+ },
+ {
+ "name": "16 Eta Sagittae",
+ "pos_x": -137.40625,
+ "pos_y": -18.4375,
+ "pos_z": 80.625,
+ "stations": "MacDonald Orbital,Bounds Hub,Macgregor City,Zettel Refinery"
+ },
+ {
+ "name": "16 Librae",
+ "pos_x": 8.28125,
+ "pos_y": 62.78125,
+ "pos_z": 60.71875,
+ "stations": "Borisenko Station,Curie Port,Lu Laboratory,Keyes Silo"
+ },
+ {
+ "name": "16 Lyrae",
+ "pos_x": -113.53125,
+ "pos_y": 37.03125,
+ "pos_z": 25.53125,
+ "stations": "Hannu Ring,Budrys Ring,Svavarsson Ring,May Hub,Green Dock,Asimov Gateway,Sagan Holdings,Morris Forum,Kerr Penal colony,Ocampo's Inheritance,Crichton Observatory,Hillary Beacon"
+ },
+ {
+ "name": "16 Persei",
+ "pos_x": -61.1875,
+ "pos_y": -38.125,
+ "pos_z": -96.90625,
+ "stations": "Delbruck Hub,Stewart Gateway,Pirsan Port,Forest Beacon,Barnwell Hub"
+ },
+ {
+ "name": "16 Piscium",
+ "pos_x": -56.9375,
+ "pos_y": -83.5,
+ "pos_z": 0.53125,
+ "stations": "Hirase Platform,Haise Settlement,Rushworth Beacon,Khan Refinery,Michelson Survey"
+ },
+ {
+ "name": "16 Tau Coronae Borealis",
+ "pos_x": -65.46875,
+ "pos_y": 82.84375,
+ "pos_z": 40.53125,
+ "stations": "Karlsefni Hub,Lem Dock,la Cosa Port,Scithers Ring,Reed Ring,McDaniel Terminal,Bass Orbital"
+ },
+ {
+ "name": "169 G. Canis Majoris",
+ "pos_x": 88.125,
+ "pos_y": 3,
+ "pos_z": -75.71875,
+ "stations": "Bond Dock"
+ },
+ {
+ "name": "17 Beta Piscis Austrini",
+ "pos_x": -18.65625,
+ "pos_y": -123.59375,
+ "pos_z": 69.09375,
+ "stations": "McKie Station,Proctor Vision,Kreutz Vista"
+ },
+ {
+ "name": "17 Crateris",
+ "pos_x": 73,
+ "pos_y": 44.125,
+ "pos_z": 17.625,
+ "stations": "Delbruck Dock,Al-Jazari Port,Runco Terminal,Lichtenberg Station"
+ },
+ {
+ "name": "17 Cygni",
+ "pos_x": -64.53125,
+ "pos_y": 5,
+ "pos_z": 24.59375,
+ "stations": "Ahern Holdings,Kuttner Point,Grant Station,Blackman Station,Krylov Station,Hassanein Vision,Lloyd Base"
+ },
+ {
+ "name": "17 Draconis",
+ "pos_x": -305.75,
+ "pos_y": 272.21875,
+ "pos_z": 49.5,
+ "stations": "Paradiso Outpost"
+ },
+ {
+ "name": "17 Kappa-1 Bootis",
+ "pos_x": -73.28125,
+ "pos_y": 132.375,
+ "pos_z": -7.34375,
+ "stations": "Grimwood Enterprise"
+ },
+ {
+ "name": "17 Lyrae",
+ "pos_x": -119.90625,
+ "pos_y": 25.375,
+ "pos_z": 58.0625,
+ "stations": "Langford Enterprise,Cady Terminal,Alpers Station,Martins Installation,Ross Gateway,Cole Station,Lambert Ring,Mourelle Terminal,Linaweaver Base,Matheson Mines,Minkowski Enterprise,Arrhenius Depot"
+ },
+ {
+ "name": "17 Theta Sagittae",
+ "pos_x": -128.09375,
+ "pos_y": -18.09375,
+ "pos_z": 71.03125,
+ "stations": "Kornbluth Prospect,Burckhardt Port"
+ },
+ {
+ "name": "17 Virginis",
+ "pos_x": 36.625,
+ "pos_y": 89.84375,
+ "pos_z": 10.4375,
+ "stations": "Nagel Outpost,Hodgkin Platform"
+ },
+ {
+ "name": "171 G. Aquarii",
+ "pos_x": -64.15625,
+ "pos_y": -87.90625,
+ "pos_z": 29.53125,
+ "stations": "Elcano Dock,Weber Landing,Filter Vision"
+ },
+ {
+ "name": "18 Aquarii",
+ "pos_x": -74.3125,
+ "pos_y": -99.09375,
+ "pos_z": 90.90625,
+ "stations": "Filipchenko Relay,Richards Terminal"
+ },
+ {
+ "name": "18 Ceti",
+ "pos_x": -22.09375,
+ "pos_y": -97.875,
+ "pos_z": -12.5625,
+ "stations": "Landsteiner City,Bella City,Lovell Dock,Soukup Keep,Lowry Vision"
+ },
+ {
+ "name": "18 e Ursae Majoris",
+ "pos_x": -24.625,
+ "pos_y": 80.03125,
+ "pos_z": -81.5625,
+ "stations": "Stefansson Outpost"
+ },
+ {
+ "name": "18 Lambda Piscium",
+ "pos_x": -58.59375,
+ "pos_y": -89.09375,
+ "pos_z": -1.46875,
+ "stations": "Hausdorff Hub,Barnaby Station,Nylund Gateway,Foden Gateway,Konscak Penal colony"
+ },
+ {
+ "name": "18 Puppis",
+ "pos_x": 58.6875,
+ "pos_y": 13.9375,
+ "pos_z": -41.125,
+ "stations": "Kraepelin City,Shatalov Station,Moore Terminal,Boulle Observatory,Willis Relay"
+ },
+ {
+ "name": "18 Scorpii",
+ "pos_x": -3.53125,
+ "pos_y": 21.65625,
+ "pos_z": 39.65625,
+ "stations": "Swift Orbital,Walters Station,Alvarez de Pineda City,Bolger Gateway,Laird Orbital,Eschbach Installation,Fadlan Ring,Dashiell Landing,de Caminha Keep,Carrasco's Claim,Kratman Horizons,Normand Works"
+ },
+ {
+ "name": "19 Aquilae",
+ "pos_x": -97.34375,
+ "pos_y": -4.21875,
+ "pos_z": 113.1875,
+ "stations": "Chretien Port,Scobee Landing,Raleigh Vista"
+ },
+ {
+ "name": "19 Leonis Minoris",
+ "pos_x": 0.3125,
+ "pos_y": 72.8125,
+ "pos_z": -55.90625,
+ "stations": "Tshang City,Moffitt Terminal,Beaumont Terminal,Litke City,Alexander Terminal,Mitchison Orbital,Huxley Port,Atwood Terminal,Arnason Hub"
+ },
+ {
+ "name": "19 Phi-2 Ceti",
+ "pos_x": -12.3125,
+ "pos_y": -49.21875,
+ "pos_z": -8.28125,
+ "stations": "Crick City,Vishweswarayya Ring,Culbertson Terminal,Ricci Landing,Zindell Base,Garriott Hub,Borisenko Gateway,Corte-Real Terminal,Baturin Gateway,Leibniz Dock,Chargaff Point"
+ },
+ {
+ "name": "19 Xi Coronae Borealis",
+ "pos_x": -102.53125,
+ "pos_y": 126.90625,
+ "pos_z": 83.84375,
+ "stations": "Shawl Platform,Shepherd Port,Peary Refinery"
+ },
+ {
+ "name": "2 Hydrae",
+ "pos_x": 115.09375,
+ "pos_y": 54.375,
+ "pos_z": -102.0625,
+ "stations": "Vardeman Base,Tall Landing"
+ },
+ {
+ "name": "2 Lyncis",
+ "pos_x": -60.40625,
+ "pos_y": 52.59375,
+ "pos_z": -134.5625,
+ "stations": "Schilling Settlement,Saunders Colony,Haldeman II Relay,Van Scyoc Prospect"
+ },
+ {
+ "name": "2 Omega Leonis",
+ "pos_x": 60.59375,
+ "pos_y": 71.21875,
+ "pos_z": -62.125,
+ "stations": "Tryggvason Terminal,Creamer Station,Edwards City,Paulo da Gama Palace,Macquorn Rankine Settlement"
+ },
+ {
+ "name": "20 Arietis",
+ "pos_x": -63.9375,
+ "pos_y": -74.53125,
+ "pos_z": -96.03125,
+ "stations": "Hirase Colony"
+ },
+ {
+ "name": "20 Leonis Minoris",
+ "pos_x": 7.78125,
+ "pos_y": 39.40625,
+ "pos_z": -28.1875,
+ "stations": "Poncelet Terminal"
+ },
+ {
+ "name": "20 Ophiuchi",
+ "pos_x": -14.28125,
+ "pos_y": 36.1875,
+ "pos_z": 96.75,
+ "stations": "Chargaff Port,Artsebarsky Gateway,Walters Silo"
+ },
+ {
+ "name": "21 Arietis",
+ "pos_x": -76.28125,
+ "pos_y": -92.0625,
+ "pos_z": -116.03125,
+ "stations": "Descartes Landing"
+ },
+ {
+ "name": "21 Draco",
+ "pos_x": -60.03125,
+ "pos_y": 45.65625,
+ "pos_z": 8.09375,
+ "stations": "Chilton Terminal,Davis Enterprise,Atwater Terminal,Dunn Orbital,Still Dock,Scott Orbital,Onizuka Station"
+ },
+ {
+ "name": "21 Eta Cygni",
+ "pos_x": -127.6875,
+ "pos_y": 7.3125,
+ "pos_z": 43.125,
+ "stations": "Fincke Landing"
+ },
+ {
+ "name": "21 Iota Serpentis",
+ "pos_x": -63.90625,
+ "pos_y": 145.375,
+ "pos_z": 104.40625,
+ "stations": "Lerner Prospect,Herbert Observatory"
+ },
+ {
+ "name": "21 Leonis Minoris",
+ "pos_x": 9.0625,
+ "pos_y": 75.3125,
+ "pos_z": -52.25,
+ "stations": "von Bellingshausen Orbital,Thagard Survey,Barcelos Hub"
+ },
+ {
+ "name": "22 g Leonis",
+ "pos_x": 39.25,
+ "pos_y": 103.96875,
+ "pos_z": -77.03125,
+ "stations": "Banks Dock"
+ },
+ {
+ "name": "22 Gamma Piscis Austrini",
+ "pos_x": -21.90625,
+ "pos_y": -194.15625,
+ "pos_z": 90.75,
+ "stations": "Maupertuis Settlement,Stillman Beacon,Piazzi Platform"
+ },
+ {
+ "name": "23 Andromedae",
+ "pos_x": -95.625,
+ "pos_y": -40.9375,
+ "pos_z": -46.3125,
+ "stations": "Amis Orbital,Vonnegut Hub,Hinz Station,Tshang Hub,Andersson Relay,Lamarck Silo,de Andrade Laboratory,Lebesgue Orbital,Whymper Vista"
+ },
+ {
+ "name": "23 Arietis",
+ "pos_x": -40.71875,
+ "pos_y": -64.4375,
+ "pos_z": -71.375,
+ "stations": "Stefansson Settlement,Collins Works,Lerner Landing,Wilmore Depot"
+ },
+ {
+ "name": "23 Delta Piscis Austrini",
+ "pos_x": -15.96875,
+ "pos_y": -139.78125,
+ "pos_z": 63,
+ "stations": "Obruchev Vision,Almagro Prospect,Weinbaum Installation"
+ },
+ {
+ "name": "23 h Ursae Majoris",
+ "pos_x": -28.1875,
+ "pos_y": 52.1875,
+ "pos_z": -50.15625,
+ "stations": "Ellern Station,Herzfeld Survey"
+ },
+ {
+ "name": "23 Librae",
+ "pos_x": 26.46875,
+ "pos_y": 38.59375,
+ "pos_z": 71.5,
+ "stations": "Howe Relay"
+ },
+ {
+ "name": "233 G. Aquarii",
+ "pos_x": -49.1875,
+ "pos_y": -191.59375,
+ "pos_z": 49.84375,
+ "stations": "Bode's Claim"
+ },
+ {
+ "name": "234 G. Carinae",
+ "pos_x": 93.71875,
+ "pos_y": -21.96875,
+ "pos_z": 41.375,
+ "stations": "Rand Orbital,Cartan Terminal,Watts Dock,Mitropoulos Enterprise,Barbaro Installation,Bode Installation,Vasilyev Observatory"
+ },
+ {
+ "name": "239 G. Aquarii",
+ "pos_x": -75.21875,
+ "pos_y": -134.0625,
+ "pos_z": 13.46875,
+ "stations": "Schottky Enterprise,Amis Oasis,Herreshoff Landing"
+ },
+ {
+ "name": "24 Aquarii",
+ "pos_x": -94.875,
+ "pos_y": -86.3125,
+ "pos_z": 64.1875,
+ "stations": "Ziemianski Port,Hedin Holdings"
+ },
+ {
+ "name": "24 Canum Venaticorum",
+ "pos_x": -69.3125,
+ "pos_y": 165.5625,
+ "pos_z": -17.21875,
+ "stations": "Elcano Platform,Hillary Lab"
+ },
+ {
+ "name": "24 G. Carinae",
+ "pos_x": 164.03125,
+ "pos_y": -65.375,
+ "pos_z": -1.96875,
+ "stations": "Knight Survey,Chawla Landing"
+ },
+ {
+ "name": "24 Iota Crateris",
+ "pos_x": 60,
+ "pos_y": 62.65625,
+ "pos_z": 8.375,
+ "stations": "Haber Dock,Cartan Terminal"
+ },
+ {
+ "name": "241 G. Aquarii",
+ "pos_x": -41.78125,
+ "pos_y": -73.96875,
+ "pos_z": 6.46875,
+ "stations": "d'Eyncourt Oasis,Gibson City,Anderson Ring,Romanenko Enterprise,Nearchus Hub,Kronecker Beacon,Santos Enterprise"
+ },
+ {
+ "name": "25 Arietis",
+ "pos_x": -30.875,
+ "pos_y": -86.625,
+ "pos_z": -79.40625,
+ "stations": "Carrasco Settlement,Baker Orbital,Kwolek Platform,Lazutkin Orbital"
+ },
+ {
+ "name": "25 Canum Venaticorum",
+ "pos_x": -45.4375,
+ "pos_y": 193.0625,
+ "pos_z": 10.53125,
+ "stations": "Macgregor Legacy"
+ },
+ {
+ "name": "25 d2 Cancri",
+ "pos_x": 59.78125,
+ "pos_y": 70.78125,
+ "pos_z": -113.59375,
+ "stations": "Doi Station,Lysenko Dock,Bosch Enterprise"
+ },
+ {
+ "name": "25 G. Canis Minoris",
+ "pos_x": 68.34375,
+ "pos_y": 29.34375,
+ "pos_z": -104.4375,
+ "stations": "Abasheli Terminal,Hume Escape"
+ },
+ {
+ "name": "25 Nu-2 Draconis",
+ "pos_x": -82.8125,
+ "pos_y": 54.125,
+ "pos_z": 10.1875,
+ "stations": "Avicenna City,Tucker Survey"
+ },
+ {
+ "name": "26 Alpha Monocerotis",
+ "pos_x": 108.28125,
+ "pos_y": 17.9375,
+ "pos_z": -98.96875,
+ "stations": "Hennepin Enterprise,Nehsi Hub,Hinz Dock,Hill Holdings,Kennan Silo"
+ },
+ {
+ "name": "26 Ceti",
+ "pos_x": -72.125,
+ "pos_y": -171.5625,
+ "pos_z": -61.9375,
+ "stations": "Kier Colony"
+ },
+ {
+ "name": "26 Draconis",
+ "pos_x": -39,
+ "pos_y": 24.90625,
+ "pos_z": -0.65625,
+ "stations": "Veach Plant,Luiken's Folly"
+ },
+ {
+ "name": "26 Ophiuchi",
+ "pos_x": 3.875,
+ "pos_y": 19.0625,
+ "pos_z": 108.1875,
+ "stations": "Locke Market,Roentgen Ring,Agnesi Silo,Readdy Gateway,Bogdanov Prospect,Greenland's Progress,Armstrong Gateway"
+ },
+ {
+ "name": "27 b1 Cygni",
+ "pos_x": -74.75,
+ "pos_y": 2.5,
+ "pos_z": 22.5625,
+ "stations": "Sharma Platform,Stein Survey"
+ },
+ {
+ "name": "27 G. Caeli",
+ "pos_x": 75.84375,
+ "pos_y": -62.09375,
+ "pos_z": -33.34375,
+ "stations": "Homer Hub,McDermott Vision,Weaver Terminal,Lasswitz Gateway,Forfait Holdings,Michell Hub,Whitcomb Ring,Fraunhofer Gateway,Payne-Scott Enterprise,Aldiss Bastion,Collins Camp"
+ },
+ {
+ "name": "27 Kappa Persei",
+ "pos_x": -59.40625,
+ "pos_y": -21.4375,
+ "pos_z": -93.40625,
+ "stations": "Zamka City,Ostwald Station,Clifton Relay,Bhabha Orbital,Brandenstein Terminal,Conway Port,Platt Relay,Bakewell Market,Stephenson Hub,Parmitano Enterprise,Scully-Power Station,Lawson Hub,Burgess Prospect"
+ },
+ {
+ "name": "28 Beta Serpentis",
+ "pos_x": -46.28125,
+ "pos_y": 113.96875,
+ "pos_z": 94.4375,
+ "stations": "Diophantus Station,Darnielle Hub,Robinson Installation"
+ },
+ {
+ "name": "28 Doradus",
+ "pos_x": 194.53125,
+ "pos_y": -125.125,
+ "pos_z": 12.75,
+ "stations": "Haro Port,Stephan Enterprise,Humphreys Refinery"
+ },
+ {
+ "name": "28 Omega Piscium",
+ "pos_x": -60.46875,
+ "pos_y": -84.09375,
+ "pos_z": -12.71875,
+ "stations": "Hassanein Dock"
+ },
+ {
+ "name": "29 Arietis",
+ "pos_x": -29.09375,
+ "pos_y": -63.625,
+ "pos_z": -68.15625,
+ "stations": "Buckland Station,Glenn Enterprise,Coats Station"
+ },
+ {
+ "name": "29 Ceti",
+ "pos_x": -57.75,
+ "pos_y": -137.28125,
+ "pos_z": -53.125,
+ "stations": "Crown Port,Rusch Dock,Amis Base"
+ },
+ {
+ "name": "29 e Orionis",
+ "pos_x": 73.1875,
+ "pos_y": -60.03125,
+ "pos_z": -125.6875,
+ "stations": "Gibbs Silo"
+ },
+ {
+ "name": "2MASS J03291977+3124572",
+ "pos_x": -379.8125,
+ "pos_y": -382.09375,
+ "pos_z": -954.46875,
+ "stations": "Ring Mine"
+ },
+ {
+ "name": "3 Beta Lacertae",
+ "pos_x": -166.03125,
+ "pos_y": -12.625,
+ "pos_z": -34.21875,
+ "stations": "Stiegler Terminal,Griffin Survey,Bixby's Folly"
+ },
+ {
+ "name": "3 Corvi",
+ "pos_x": 139.875,
+ "pos_y": 118.59375,
+ "pos_z": 56.3125,
+ "stations": "Lichtenberg Dock,Herbert Dock,Quimper Camp"
+ },
+ {
+ "name": "3 Upsilon Ophiuchi",
+ "pos_x": -13.5625,
+ "pos_y": 53.875,
+ "pos_z": 108.9375,
+ "stations": "Patterson Depot,Hendel Station,Maury Survey"
+ },
+ {
+ "name": "30 Ceti",
+ "pos_x": -32.09375,
+ "pos_y": -146.125,
+ "pos_z": -35.625,
+ "stations": "Schiaparelli Vision,Qureshi Laboratory,Lopez de Villalobos Landing,Quaglia Base"
+ },
+ {
+ "name": "30 Lyncis",
+ "pos_x": -29.75,
+ "pos_y": 60.46875,
+ "pos_z": -81.21875,
+ "stations": "Euclid Colony"
+ },
+ {
+ "name": "30 Serpentis",
+ "pos_x": -10.96875,
+ "pos_y": 105,
+ "pos_z": 141.0625,
+ "stations": "Chios' Folly,Webb Vision,Weinbaum Arena"
+ },
+ {
+ "name": "31 Aquilae",
+ "pos_x": -36.625,
+ "pos_y": -2.03125,
+ "pos_z": 33.25,
+ "stations": "Ingstad Enterprise,Compton Hub,Nearchus Hub,Svavarsson Landing,Isherwood Gateway,Russo Dock,Killough Orbital,Hannu Dock,Laird Gateway,Zoline Base,Roberts Installation,Xuanzang's Folly,Merchiston Terminal"
+ },
+ {
+ "name": "31 Arietis",
+ "pos_x": -29,
+ "pos_y": -76.375,
+ "pos_z": -78.53125,
+ "stations": "Ham Enterprise,Cooper Relay,Quimby Platform"
+ },
+ {
+ "name": "31 Beta Leonis Minoris",
+ "pos_x": 9.1875,
+ "pos_y": 131.75,
+ "pos_z": -79.03125,
+ "stations": "Cenker Base,Volynov Colony,Seddon Reach"
+ },
+ {
+ "name": "31 Cephei",
+ "pos_x": -159.25,
+ "pos_y": 41.625,
+ "pos_z": -70.40625,
+ "stations": "Selous Landing,Zoline Port,Giles Camp"
+ },
+ {
+ "name": "31 Epsilon Librae",
+ "pos_x": 9.53125,
+ "pos_y": 60.9375,
+ "pos_z": 81.0625,
+ "stations": "Dorsey Terminal,Steinmuller Dock,Levy Base,Mieville Port,Sleator's Folly"
+ },
+ {
+ "name": "31 Vulpeculae",
+ "pos_x": -175.65625,
+ "pos_y": -36.75,
+ "pos_z": 57.875,
+ "stations": "Erdos Relay"
+ },
+ {
+ "name": "32 c Piscium",
+ "pos_x": -71.3125,
+ "pos_y": -95.34375,
+ "pos_z": -17.71875,
+ "stations": "Eyharts Enterprise,Herrington Enterprise,Cayley Orbital,Pennington Exchange,Rescue Ship - Eyharts Enterprise"
+ },
+ {
+ "name": "32 l Persei",
+ "pos_x": -73.40625,
+ "pos_y": -28.96875,
+ "pos_z": -128.28125,
+ "stations": "Jeury Station,Simak Enterprise"
+ },
+ {
+ "name": "32 Mu Serpentis",
+ "pos_x": -11.75,
+ "pos_y": 101.3125,
+ "pos_z": 135.5,
+ "stations": "Herodotus Dock,O'Leary Terminal,Eckford Point"
+ },
+ {
+ "name": "33 Bootis",
+ "pos_x": -84.75,
+ "pos_y": 164.53125,
+ "pos_z": 18,
+ "stations": "Schouten Hub,Minkowski Vision"
+ },
+ {
+ "name": "33 Cygni",
+ "pos_x": -155.75,
+ "pos_y": 33.09375,
+ "pos_z": -3.53125,
+ "stations": "Cremona Enterprise,Gurney Beacon"
+ },
+ {
+ "name": "33 G. Antlia",
+ "pos_x": 162.375,
+ "pos_y": 62.5,
+ "pos_z": -23.8125,
+ "stations": "Peano Platform,Phillips Vista"
+ },
+ {
+ "name": "33 G. Canis Majoris",
+ "pos_x": 75.9375,
+ "pos_y": -26.96875,
+ "pos_z": -54.75,
+ "stations": "Lethem Vision,Abasheli Base,Malzberg Terminal,Wiley Dock,Stackpole Escape,Chiang Silo"
+ },
+ {
+ "name": "33 Sextantis",
+ "pos_x": 76.59375,
+ "pos_y": 88.78125,
+ "pos_z": -25.90625,
+ "stations": "Murray Gateway,Crippen Port,Sacco Orbital,Padalka City,Covey Hub,Czerneda Installation,Chasles Gateway,Ryman Vision,Tuan Beacon"
+ },
+ {
+ "name": "33 Theta Cassiopeiae",
+ "pos_x": -106.9375,
+ "pos_y": -17.03125,
+ "pos_z": -78.21875,
+ "stations": "Kekule Landing,Serrao Vision"
+ },
+ {
+ "name": "34 Omicron Cephei",
+ "pos_x": -183.15625,
+ "pos_y": 24.65625,
+ "pos_z": -84.25,
+ "stations": "Wilson Port"
+ },
+ {
+ "name": "34 Pegasi",
+ "pos_x": -85.375,
+ "pos_y": -85.1875,
+ "pos_z": 30.65625,
+ "stations": "Weiss Orbital,Webb Port,Rayhan al-Biruni Orbital,Williams City,Henderson Dock,Ozanne Vision,Marques Forum,Morris Vision,Clark Keep,Shukor Keep,Archimedes Holdings,Frobisher City"
+ },
+ {
+ "name": "34 Theta Geminorum",
+ "pos_x": 7.75,
+ "pos_y": 50.90625,
+ "pos_z": -181.9375,
+ "stations": "Noah's Rest"
+ },
+ {
+ "name": "35 Ceti",
+ "pos_x": -56.625,
+ "pos_y": -135.65625,
+ "pos_z": -55.96875,
+ "stations": "Farrer Landing,Cassidy Enterprise,Nusslein-Volhard Horizons"
+ },
+ {
+ "name": "35 Draconis",
+ "pos_x": -85.125,
+ "pos_y": 51.75,
+ "pos_z": -28.375,
+ "stations": "Conway Dock,Nelson Depot,Normand Enterprise"
+ },
+ {
+ "name": "35 Leonis",
+ "pos_x": 29.4375,
+ "pos_y": 83.78125,
+ "pos_z": -49.5,
+ "stations": "Oleskiw Gateway,Merle Base,Potrykus City,Blaha Hub"
+ },
+ {
+ "name": "35 Leonis Minoris",
+ "pos_x": 9.25,
+ "pos_y": 136.3125,
+ "pos_z": -76.1875,
+ "stations": "Zholobov Settlement,Bondar Enterprise,Chern Settlement"
+ },
+ {
+ "name": "36 Andromedae",
+ "pos_x": -79.15625,
+ "pos_y": -77.90625,
+ "pos_z": -54.9375,
+ "stations": "Herzfeld Terminal,Heisenberg Ring,Dyson Station"
+ },
+ {
+ "name": "36 Cygni",
+ "pos_x": -197.28125,
+ "pos_y": 1.4375,
+ "pos_z": 51.40625,
+ "stations": "Hiroyuki Reach"
+ },
+ {
+ "name": "36 Doradus",
+ "pos_x": 73.78125,
+ "pos_y": -43.3125,
+ "pos_z": 3.03125,
+ "stations": "Wang Port,Pudwill Gorie Dock,Guidoni Port,Coulomb Landing,Dyson Terminal,Hadfield Station,Stephenson Terminal,Mukai Holdings,Tryggvason Base,Gardner Dock,Al-Jazari City,Shargin Dock"
+ },
+ {
+ "name": "36 Ophiuchi",
+ "pos_x": 0.4375,
+ "pos_y": 2.09375,
+ "pos_z": 19.21875,
+ "stations": "Yolen Works,Watts Stop,Weinbaum City,Katzenstein Dock,Lunan Holdings"
+ },
+ {
+ "name": "36 Persei",
+ "pos_x": -58.25,
+ "pos_y": -15.96875,
+ "pos_z": -101.96875,
+ "stations": "Abasheli Ring,Herreshoff Hub,Rodrigues Dock,Busch Port,Fisher Terminal,Alexander Terminal,Toll Camp,Mitra Terminal,Rolland Barracks"
+ },
+ {
+ "name": "36 Ursae Majoris",
+ "pos_x": -11.125,
+ "pos_y": 32.9375,
+ "pos_z": -23,
+ "stations": "Aristotle Platform"
+ },
+ {
+ "name": "37 Geminorum",
+ "pos_x": 10.25,
+ "pos_y": 12.3125,
+ "pos_z": -53.90625,
+ "stations": "Bombelli Landing,Hinz Station,Chasles Port,Grant Gateway,Stairs Terminal,Frechet Ring,Utley City,Bugrov Legacy,Carstensz Laboratory,Barcelos Bastion,Perga Gateway,Davidson Hub,Vlamingh Vision"
+ },
+ {
+ "name": "37 Librae",
+ "pos_x": 5.8125,
+ "pos_y": 54.375,
+ "pos_z": 76.875,
+ "stations": "den Berg Gateway,Roosa Market,Kovalyonok Enterprise,Ross Installation,King Holdings"
+ },
+ {
+ "name": "37 Lyncis",
+ "pos_x": -14.65625,
+ "pos_y": 62.6875,
+ "pos_z": -62.21875,
+ "stations": "Rorschach Ring,Disch Vision,Barentsz Arsenal"
+ },
+ {
+ "name": "37 Ursae Majoris",
+ "pos_x": -24.84375,
+ "pos_y": 68.21875,
+ "pos_z": -47.03125,
+ "stations": "Oppenheimer Dock,Crichton Depot"
+ },
+ {
+ "name": "37 Xi Bootis",
+ "pos_x": -4.21875,
+ "pos_y": 19.09375,
+ "pos_z": 9.8125,
+ "stations": "Bresnik Terminal"
+ },
+ {
+ "name": "38 Arietis",
+ "pos_x": -27.5625,
+ "pos_y": -78.125,
+ "pos_z": -84.78125,
+ "stations": "Nelson Point,Moore Works"
+ },
+ {
+ "name": "38 Leonis Minoris",
+ "pos_x": 5.125,
+ "pos_y": 149.125,
+ "pos_z": -82.875,
+ "stations": "Murphy City,Maine Landing,Fawcett Keep,Grassmann Works"
+ },
+ {
+ "name": "38 Lyncis",
+ "pos_x": 10.96875,
+ "pos_y": 88.375,
+ "pos_z": -87.46875,
+ "stations": "Ozanne Installation,MacLeod Market,Corte-Real Arsenal,Zillig Base"
+ },
+ {
+ "name": "38 Virginis",
+ "pos_x": 44.96875,
+ "pos_y": 91.53125,
+ "pos_z": 31.53125,
+ "stations": "Bamford Ring,Balandin Hub,Burnell Colony,Norgay Survey"
+ },
+ {
+ "name": "39 Aquarii",
+ "pos_x": -60.5,
+ "pos_y": -107.0625,
+ "pos_z": 60.78125,
+ "stations": "Riemann Installation,Thierree Mine"
+ },
+ {
+ "name": "39 Arietis",
+ "pos_x": -72.1875,
+ "pos_y": -76.84375,
+ "pos_z": -135.375,
+ "stations": "Houtman Terminal,MacLeod's Progress,Nourse Enterprise"
+ },
+ {
+ "name": "39 b Draconis",
+ "pos_x": -164.9375,
+ "pos_y": 81.75,
+ "pos_z": 5.96875,
+ "stations": "Alvares Settlement"
+ },
+ {
+ "name": "39 Herculis",
+ "pos_x": -81.0625,
+ "pos_y": 89.3125,
+ "pos_z": 76.21875,
+ "stations": "Tudela City,Petaja Vision,Hausdorff Vista"
+ },
+ {
+ "name": "39 Leonis",
+ "pos_x": 21.96875,
+ "pos_y": 61.3125,
+ "pos_z": -35.875,
+ "stations": "Baxter Landing,Wegener Dock,Cabana Terminal,Runco City,Gamow Horizons,Walheim Enterprise,Khayyam City,Lucretius Dock,Sherrington Dock,Balmer's Inheritance,Cugnot Dock,Shumil Terminal,Brady City,Menezes Prospect"
+ },
+ {
+ "name": "39 Serpentis",
+ "pos_x": -16.46875,
+ "pos_y": 39.875,
+ "pos_z": 36.59375,
+ "stations": "Adamson Terminal,Rushd Ring,Lindsey Orbital"
+ },
+ {
+ "name": "39 Tauri",
+ "pos_x": -7.3125,
+ "pos_y": -20.28125,
+ "pos_z": -50.90625,
+ "stations": "Porta"
+ },
+ {
+ "name": "4 A1 Virginis",
+ "pos_x": 77.875,
+ "pos_y": 176.3125,
+ "pos_z": -9.40625,
+ "stations": "Czerneda's Pride,Nehsi Prospect,Gold Legacy,Aikin Survey"
+ },
+ {
+ "name": "4 Camelopardalis",
+ "pos_x": -81.15625,
+ "pos_y": 24.03125,
+ "pos_z": -149.90625,
+ "stations": "Song Station,Laval Dock,Low Station,Ibold Installation,Tanaka Terminal"
+ },
+ {
+ "name": "4 Eridani",
+ "pos_x": 41.5,
+ "pos_y": -135.9375,
+ "pos_z": -63.1875,
+ "stations": "Goldberg Platform,Fowler Station,Gent Installation,Garfinkle Landing"
+ },
+ {
+ "name": "4 Pegasi",
+ "pos_x": -130.96875,
+ "pos_y": -98,
+ "pos_z": 71.28125,
+ "stations": "Butcher Forum"
+ },
+ {
+ "name": "4 Sextantis",
+ "pos_x": 87.25,
+ "pos_y": 96.84375,
+ "pos_z": -65,
+ "stations": "Rukavishnikov Hangar"
+ },
+ {
+ "name": "40 Ceti",
+ "pos_x": -27.96875,
+ "pos_y": -87.59375,
+ "pos_z": -32.3125,
+ "stations": "Pailes Enterprise,Illy Port,Andersson Terminal"
+ },
+ {
+ "name": "40 Draconis",
+ "pos_x": -134.78125,
+ "pos_y": 80.6875,
+ "pos_z": -53.9375,
+ "stations": "Capek City,Greenland City,Sohl Port,Sharp Camp,Shelley Settlement"
+ },
+ {
+ "name": "40 Leonis",
+ "pos_x": 24.5625,
+ "pos_y": 57.0625,
+ "pos_z": -31.5625,
+ "stations": "Archer Relay,Goeschke Horizons"
+ },
+ {
+ "name": "40 Leonis Minoris",
+ "pos_x": 32.5625,
+ "pos_y": 130.84375,
+ "pos_z": -61.625,
+ "stations": "Sagan Dock,Brule Settlement,Karlsefni Silo"
+ },
+ {
+ "name": "41 Gamma Serpentis",
+ "pos_x": -12.125,
+ "pos_y": 26,
+ "pos_z": 22.875,
+ "stations": "Kidman Settlement,Boe Depot,Bolotov Port,Forest Refinery,Teng Horizons"
+ },
+ {
+ "name": "41 Lambda Hydrae",
+ "pos_x": 88.78125,
+ "pos_y": 64.09375,
+ "pos_z": -25.90625,
+ "stations": "Readdy City,Yurchikhin Ring,Conrad Enterprise,Gamow Terminal,Hartlib Station,Liska Orbital,Scully-Power Ring,Nowak Dock,Chilton Orbital,Laird Hub"
+ },
+ {
+ "name": "41 Upsilon-4 Eridani",
+ "pos_x": 102.375,
+ "pos_y": -126,
+ "pos_z": -72.875,
+ "stations": "Flagg Station,Filippenko Ring,Tomita Vision,Stephan Gateway,Wright Station,Williams Installation"
+ },
+ {
+ "name": "42 Aquilae",
+ "pos_x": -57.84375,
+ "pos_y": -23.53125,
+ "pos_z": 84.25,
+ "stations": "Caidin Town,Caselberg Keep,Born Station,Ride Market,Locke Station,Aldrin Horizons,Makarov Dock,Duke Enterprise,Fullerton Hub,Baker Hub,Crippen Terminal,Chretien Orbital"
+ },
+ {
+ "name": "42 Capricorni",
+ "pos_x": -49.96875,
+ "pos_y": -75.96875,
+ "pos_z": 59.03125,
+ "stations": "Lonchakov Holdings,Ross City"
+ },
+ {
+ "name": "42 n Persei",
+ "pos_x": -83.5625,
+ "pos_y": -73.40625,
+ "pos_z": -244.34375,
+ "stations": "PRE Logistics Support Alpha,Bennington's Rest,Rescue Ship - Bennington's Rest"
+ },
+ {
+ "name": "44 b Ophiuchi",
+ "pos_x": -3,
+ "pos_y": 8.0625,
+ "pos_z": 82.6875,
+ "stations": "Feustel Gateway,Rotsler Enterprise"
+ },
+ {
+ "name": "44 chi Draconis",
+ "pos_x": -22.5625,
+ "pos_y": 12.375,
+ "pos_z": -5.40625,
+ "stations": "Steinmuller City"
+ },
+ {
+ "name": "44 Eta Librae",
+ "pos_x": 15.90625,
+ "pos_y": 73.53125,
+ "pos_z": 128.8125,
+ "stations": "Moisuc Platform"
+ },
+ {
+ "name": "44 G. Aquilae",
+ "pos_x": -87.59375,
+ "pos_y": -6.0625,
+ "pos_z": 85.46875,
+ "stations": "Polansky Settlement,Bamford Mines,Khrunov Relay"
+ },
+ {
+ "name": "44 Pi Serpentis",
+ "pos_x": -76.46875,
+ "pos_y": 129.34375,
+ "pos_z": 97.3125,
+ "stations": "Danvers Survey,Carlisle Terminal,Alpers Oasis,Fu Barracks"
+ },
+ {
+ "name": "44 Rho-1 Sagittarii",
+ "pos_x": -42.8125,
+ "pos_y": -33.15625,
+ "pos_z": 114.8125,
+ "stations": "Crippen Reach,Pauling Port,Davy Settlement"
+ },
+ {
+ "name": "45 c Bootis",
+ "pos_x": -19.46875,
+ "pos_y": 54.625,
+ "pos_z": 26.5,
+ "stations": "Ride Hub,Kepler Hub"
+ },
+ {
+ "name": "45 d Ophiuchi",
+ "pos_x": 5,
+ "pos_y": 4.375,
+ "pos_z": 111.375,
+ "stations": "Hire Orbital,Tito Beacon,Arber Port,Dunn Dock,Oltion Works"
+ },
+ {
+ "name": "45 Tauri",
+ "pos_x": 12.4375,
+ "pos_y": -62.34375,
+ "pos_z": -102.53125,
+ "stations": "Matheson Prospect,Herrington Gateway"
+ },
+ {
+ "name": "45 Theta Ceti",
+ "pos_x": -21.59375,
+ "pos_y": -106.3125,
+ "pos_z": -34.46875,
+ "stations": "Goddard Ring,Swainson City,Bhabha Gateway,Walheim Orbital,Olivas Station,Otto Terminal,Lovell Holdings,Gaspar de Portola Keep,Oleskiw Station,Howard Observatory"
+ },
+ {
+ "name": "47 Arietis",
+ "pos_x": -32.03125,
+ "pos_y": -58.4375,
+ "pos_z": -85.25,
+ "stations": "Crown Station"
+ },
+ {
+ "name": "47 Cassiopeiae",
+ "pos_x": -83.03125,
+ "pos_y": 28.5625,
+ "pos_z": -63.1875,
+ "stations": "Hurston Orbital,Agassiz Orbital,Virchow City,Archimedes Enterprise,Gillekens Gateway,Tuan Enterprise,Meitner Terminal,Mitchell Hub,Broglie Beacon,Glidden City"
+ },
+ {
+ "name": "47 Ceti",
+ "pos_x": -14.125,
+ "pos_y": -116.96875,
+ "pos_z": -32.53125,
+ "stations": "Kaufmanis Hub,Glushko Station,Argelander Gateway,Spassky Penal colony"
+ },
+ {
+ "name": "47 Ursae Majoris",
+ "pos_x": -1.4375,
+ "pos_y": 41.1875,
+ "pos_z": -20.09375,
+ "stations": "Nordenskiold Landing,Marlowe's Progress"
+ },
+ {
+ "name": "48 Tauri",
+ "pos_x": -2.4375,
+ "pos_y": -60.5,
+ "pos_z": -135.1875,
+ "stations": "Runco Landing,Surayev Beacon"
+ },
+ {
+ "name": "49 Arietis",
+ "pos_x": -74.25,
+ "pos_y": -96.53125,
+ "pos_z": -170.96875,
+ "stations": "Gaiman Dock,Landis Hub,Konscak Colony,Fisk Landing,Dirichlet Camp,Rescue Ship - Gaiman Dock"
+ },
+ {
+ "name": "49 Ceti",
+ "pos_x": -11.5,
+ "pos_y": -186.375,
+ "pos_z": -51.46875,
+ "stations": "Dillon Survey,Rosse Dock"
+ },
+ {
+ "name": "49 d Orionis",
+ "pos_x": 71.75,
+ "pos_y": -47,
+ "pos_z": -117.5,
+ "stations": "Bell Station,Musgrave Prospect,Gold's Folly,Somerset Hub,Belyayev Mines"
+ },
+ {
+ "name": "49 G. Antlia",
+ "pos_x": 98.96875,
+ "pos_y": 29.15625,
+ "pos_z": 0.34375,
+ "stations": "Cooper Orbital,Cseszneky Relay"
+ },
+ {
+ "name": "49 Serpentis",
+ "pos_x": -26.96875,
+ "pos_y": 49.96875,
+ "pos_z": 51.875,
+ "stations": "Carter Market,Ross Station,Shaw Terminal,Perrin Terminal,Andreas Terminal,Bresnik Camp,Hamilton Barracks"
+ },
+ {
+ "name": "5 Andromedae",
+ "pos_x": -105.71875,
+ "pos_y": -19.5625,
+ "pos_z": -31.4375,
+ "stations": "Lounge Station,Schrodinger Station,Faraday Orbital,Stith Oasis,al-Din Keep"
+ },
+ {
+ "name": "5 Epsilon-2 Lyrae",
+ "pos_x": -138.15625,
+ "pos_y": 47.78125,
+ "pos_z": 53.09375,
+ "stations": "Sharipov Horizons,McArthur Mine"
+ },
+ {
+ "name": "5 G. Apodis",
+ "pos_x": 71.125,
+ "pos_y": -21.03125,
+ "pos_z": 56.03125,
+ "stations": "Savery Hub,Matteucci Colony,Boyle Refinery"
+ },
+ {
+ "name": "5 G. Capricorni",
+ "pos_x": -6.96875,
+ "pos_y": -14.53125,
+ "pos_z": 24.1875,
+ "stations": "Mastracchio Orbital,Deere Dock,Moore Beacon,Euclid Dock"
+ },
+ {
+ "name": "5 Gamma Equulei",
+ "pos_x": -93.3125,
+ "pos_y": -50.28125,
+ "pos_z": 52.75,
+ "stations": "Mitropoulos Dock"
+ },
+ {
+ "name": "5 Mu Leporis",
+ "pos_x": 99.40625,
+ "pos_y": -88.4375,
+ "pos_z": -129.9375,
+ "stations": "Samos Vista"
+ },
+ {
+ "name": "5 Puppis",
+ "pos_x": 71.625,
+ "pos_y": 11.5,
+ "pos_z": -58.625,
+ "stations": "Aleksandrov Port,Curie Enterprise,Sarich Survey,Mayer Terminal,Kratman Point"
+ },
+ {
+ "name": "50 Cassiopeiae",
+ "pos_x": -120.65625,
+ "pos_y": 29,
+ "pos_z": -96.40625,
+ "stations": "Chorel Stop,Vardeman Depot"
+ },
+ {
+ "name": "50 Omega Tauri",
+ "pos_x": -7.71875,
+ "pos_y": -32.96875,
+ "pos_z": -88.125,
+ "stations": "Jernigan Prospect,Ramanujan Dock,Volta Station,Mondeh Port"
+ },
+ {
+ "name": "50 Upsilon-1 Eridani",
+ "pos_x": 73.09375,
+ "pos_y": -83.625,
+ "pos_z": -61.71875,
+ "stations": "Dzhanibekov City,Dzhanibekov Refinery,Cochrane Terminal"
+ },
+ {
+ "name": "51 Aquilae",
+ "pos_x": -43.4375,
+ "pos_y": -28.9375,
+ "pos_z": 74.4375,
+ "stations": "Bose Orbital,Jordan Hub,Boas Port,Thirsk Station,Morey City,Lunan Prospect,Avdeyev City,Coke Hub,Malerba Terminal,Carver Settlement,Mohun Landing"
+ },
+ {
+ "name": "51 Arietis",
+ "pos_x": -23.84375,
+ "pos_y": -30.78125,
+ "pos_z": -54.9375,
+ "stations": "Dillon Hub,Hippoc Bioceuticals Research Stn,Lopez de Villalobos Arsenal,Hippoc Bioceuticals Research Station"
+ },
+ {
+ "name": "51 Eridani",
+ "pos_x": 26.875,
+ "pos_y": -48.09375,
+ "pos_z": -78.625,
+ "stations": "Mendez Terminal,Grover Base,Rorschach Terminal,Pausch Ring,Springer Station,Cady Observatory,Gerlache Base"
+ },
+ {
+ "name": "51 Tauri",
+ "pos_x": -16.25,
+ "pos_y": -59.09375,
+ "pos_z": -165.34375,
+ "stations": "Rothfuss Prospect"
+ },
+ {
+ "name": "52 H2 Sagittarii",
+ "pos_x": -46,
+ "pos_y": -68.3125,
+ "pos_z": 170.8125,
+ "stations": "Steele Horizons,Van Scyoc Prospect"
+ },
+ {
+ "name": "52 Leonis Minoris",
+ "pos_x": 36.5,
+ "pos_y": 164.3125,
+ "pos_z": -58.84375,
+ "stations": "Eddington Mines"
+ },
+ {
+ "name": "52 Psi Ursae Majoris",
+ "pos_x": -15.75,
+ "pos_y": 129.65625,
+ "pos_z": -61.84375,
+ "stations": "Reis Port,Hutchinson Ring,Hiraga Terminal"
+ },
+ {
+ "name": "53 Aquarii",
+ "pos_x": -25.6875,
+ "pos_y": -54.28125,
+ "pos_z": 27.125,
+ "stations": "Yaping Terminal"
+ },
+ {
+ "name": "53 chi Ceti",
+ "pos_x": -6.6875,
+ "pos_y": -70.15625,
+ "pos_z": -27.5,
+ "stations": "Francisco de Eliza Ring,Preuss Enterprise,Andoyer Prospect,Desargues Gateway,Rawn Ring,Stevin Gateway,Mallett Orbital,Kirtley Ring,Farouk Gateway,Wirtanen Base,Caselberg Dock,Ohm Beacon"
+ },
+ {
+ "name": "53 Nu Serpentis",
+ "pos_x": -37.6875,
+ "pos_y": 45.21875,
+ "pos_z": 194.5,
+ "stations": "Hawke's Inheritance,Yolen Horizons"
+ },
+ {
+ "name": "53 Virginis",
+ "pos_x": 57.9375,
+ "pos_y": 79.59375,
+ "pos_z": 50.34375,
+ "stations": "Bennett Gateway,Sacco Gateway,Readdy Relay,Auer Prospect"
+ },
+ {
+ "name": "54 Cassiopeiae",
+ "pos_x": -67.5,
+ "pos_y": 15.28125,
+ "pos_z": -55.28125,
+ "stations": "Olivas Survey"
+ },
+ {
+ "name": "54 Ceti",
+ "pos_x": -50.0625,
+ "pos_y": -103.03125,
+ "pos_z": -75.28125,
+ "stations": "Coblentz Orbital,Fermat Ring,Sladek Gateway,Van Scyoc Port,Grzimek Dock,Fancher Landing,Binder Base,Norman Port,Lazarev Vista,Porges Hub,Henricks Holdings"
+ },
+ {
+ "name": "54 Draconis",
+ "pos_x": -155.5,
+ "pos_y": 55.75,
+ "pos_z": 3.65625,
+ "stations": "Burgess Laboratory,Huxley Oasis"
+ },
+ {
+ "name": "54 G. Antlia",
+ "pos_x": 70.375,
+ "pos_y": 24.46875,
+ "pos_z": -1.09375,
+ "stations": "Singer Dock,Ferguson Market,Hooke Terminal,Furrer City,Kondakova Station,Hopkins Dock,Wisoff Port,Alas Dock"
+ },
+ {
+ "name": "54 Piscium",
+ "pos_x": -23.46875,
+ "pos_y": -23.8125,
+ "pos_z": -13.5625,
+ "stations": "Rushd Terminal,Ampere Enterprise,Sturckow Hub"
+ },
+ {
+ "name": "55 Leonis",
+ "pos_x": 86.09375,
+ "pos_y": 115.1875,
+ "pos_z": -26.875,
+ "stations": "Artyukhin Port"
+ },
+ {
+ "name": "55 Ursae Majoris",
+ "pos_x": -3.15625,
+ "pos_y": 178.28125,
+ "pos_z": -70.78125,
+ "stations": "Le Guin Gateway"
+ },
+ {
+ "name": "55 Virginis",
+ "pos_x": 70.9375,
+ "pos_y": 85.5,
+ "pos_z": 61.6875,
+ "stations": "Gemar City,Durrance City,Qureshi Hub,Cabrera Installation,vo Terminal,Al Saud Port,Redi's Progress,Somerset Station,Zamyatin Settlement,Hottot Works,Lee Vision,Macarthur Dock"
+ },
+ {
+ "name": "55 Xi Serpentis",
+ "pos_x": -19.8125,
+ "pos_y": 14.75,
+ "pos_z": 102.34375,
+ "stations": "Mendeleev Installation,Bridges Landing"
+ },
+ {
+ "name": "56 Cygni",
+ "pos_x": -133.0625,
+ "pos_y": -0.21875,
+ "pos_z": 12.46875,
+ "stations": "Bardeen Survey"
+ },
+ {
+ "name": "57 Virginis",
+ "pos_x": 69.53125,
+ "pos_y": 84.3125,
+ "pos_z": 61.65625,
+ "stations": "Carter Vision,Coblentz's Progress,Reisman Station"
+ },
+ {
+ "name": "57 Zeta Serpentis",
+ "pos_x": -30.9375,
+ "pos_y": 12,
+ "pos_z": 69.28125,
+ "stations": "MacKellar Ring,Scortia Port,Hertz Station,Wilson Hub,Gerst Dock,Feoktistov Dock,Parmitano City,Parise Terminal,Gresh Town,Musabayev Dock,Levi-Montalcini Orbital,Williams' Inheritance"
+ },
+ {
+ "name": "58 Epsilon Herculis",
+ "pos_x": -100.3125,
+ "pos_y": 90.5625,
+ "pos_z": 75.90625,
+ "stations": "Thurston Dock,Shaara Reach,Meaney Stop"
+ },
+ {
+ "name": "58 Eridani",
+ "pos_x": 20.78125,
+ "pos_y": -24.40625,
+ "pos_z": -29.15625,
+ "stations": "Ising Port,Williams' Progress,Kelly Enterprise,Ochoa Station,Vinci Depot"
+ },
+ {
+ "name": "58 Geminorum",
+ "pos_x": 35.75,
+ "pos_y": 50.125,
+ "pos_z": -146.5625,
+ "stations": "Mohmand Hub,So-yeon Station,Jemison Orbital"
+ },
+ {
+ "name": "58 Ursae Majoris",
+ "pos_x": -19.34375,
+ "pos_y": 161.09375,
+ "pos_z": -63.4375,
+ "stations": "Dalton Vision,Al-Farabi Landing"
+ },
+ {
+ "name": "59 Eridani",
+ "pos_x": 64.71875,
+ "pos_y": -75.78125,
+ "pos_z": -92.65625,
+ "stations": "Conrad Terminal,Guidoni Dock,Wittgenstein Hub,Collins Settlement,Fraser Hub,Clement Ring,Chapman Dock,Flade Plant"
+ },
+ {
+ "name": "59 Ursae Majoris",
+ "pos_x": -19.40625,
+ "pos_y": 140.96875,
+ "pos_z": -52.3125,
+ "stations": "Teller Terminal,Oswald Colony,Parise Arsenal"
+ },
+ {
+ "name": "59 Virginis",
+ "pos_x": 10.9375,
+ "pos_y": 54.09375,
+ "pos_z": 15.25,
+ "stations": "Hilmers Gateway,Chern Point,Landsteiner Terminal,Hertz Oasis,Nourse Plant,Cantor Camp,Zettel Installation,Taine Point"
+ },
+ {
+ "name": "6 Andromedae",
+ "pos_x": -84.03125,
+ "pos_y": -24.1875,
+ "pos_z": -22.09375,
+ "stations": "Galilei Ring,Rawn Hub,Lichtenberg Landing"
+ },
+ {
+ "name": "6 Ceti",
+ "pos_x": -15.5625,
+ "pos_y": -59.125,
+ "pos_z": 1.40625,
+ "stations": "Serrao Orbital,Phillips Orbital,Lindbohm Orbital,Abnett Survey"
+ },
+ {
+ "name": "6 Lyncis",
+ "pos_x": -65.96875,
+ "pos_y": 64.375,
+ "pos_z": -156.96875,
+ "stations": "Delbruck Depot"
+ },
+ {
+ "name": "6 Zeta-1 Lyrae",
+ "pos_x": -137.4375,
+ "pos_y": 45.84375,
+ "pos_z": 58.21875,
+ "stations": "Deb Market"
+ },
+ {
+ "name": "60 b Leonis",
+ "pos_x": 37.53125,
+ "pos_y": 114.5,
+ "pos_z": -39.28125,
+ "stations": "Flade Port,Mitchison Works"
+ },
+ {
+ "name": "60 Herculis",
+ "pos_x": -63.6875,
+ "pos_y": 63.9375,
+ "pos_z": 98.09375,
+ "stations": "Furrer Port,Lazarev Hub,Love's Claim"
+ },
+ {
+ "name": "60 Tau Draconis",
+ "pos_x": -129,
+ "pos_y": 60.21875,
+ "pos_z": -34.125,
+ "stations": "Pryor Base,Okorafor Landing"
+ },
+ {
+ "name": "60 Tauri",
+ "pos_x": 2.625,
+ "pos_y": -58.375,
+ "pos_z": -132.46875,
+ "stations": "MacVicar City,Harris Enterprise,Miletus Orbital,Pond Survey,Stillman Camp"
+ },
+ {
+ "name": "61 Cygni",
+ "pos_x": -11.21875,
+ "pos_y": -1.1875,
+ "pos_z": 1.40625,
+ "stations": "Broglie Terminal,Weber Hub"
+ },
+ {
+ "name": "61 Mu Orionis",
+ "pos_x": 50.21875,
+ "pos_y": -15.46875,
+ "pos_z": -145.78125,
+ "stations": "Younghusband Dock,Lethem's Exile"
+ },
+ {
+ "name": "61 Ursae Majoris",
+ "pos_x": 0.5625,
+ "pos_y": 30.09375,
+ "pos_z": -8.6875,
+ "stations": "Boulton Hub,Dobrovolski Point,Hovgaard Market,Ibold Relay"
+ },
+ {
+ "name": "61 Virginis",
+ "pos_x": 14.8125,
+ "pos_y": 19.28125,
+ "pos_z": 13.65625,
+ "stations": "Furukawa Port,Margulis Depot,Crown Colony,Shepard Arsenal"
+ },
+ {
+ "name": "62 Gamma Ophiuchi",
+ "pos_x": -47.125,
+ "pos_y": 26.25,
+ "pos_z": 87.5,
+ "stations": "Weitz Station,Berliner Lab"
+ },
+ {
+ "name": "62 Omicron-1 Cancri",
+ "pos_x": 66.5,
+ "pos_y": 85.90625,
+ "pos_z": -102.15625,
+ "stations": "Blaschke Platform,Carson Barracks"
+ },
+ {
+ "name": "62 Ursae Majoris",
+ "pos_x": 7.59375,
+ "pos_y": 129.46875,
+ "pos_z": -34.53125,
+ "stations": "Gurney Station,Schlegel City,Akers Hub,Bolotov City,Bunch Enterprise,Alvares Installation,Gwynn's Progress,Curbeam Dock,Cartan Hub,Melnick Hub,Armstrong Orbital,Low City,Rayhan al-Biruni Installation,Tsunenaga Landing,Kozin Enterprise"
+ },
+ {
+ "name": "63 chi Leonis",
+ "pos_x": 45.90625,
+ "pos_y": 80.375,
+ "pos_z": -19.1875,
+ "stations": "Rzeppa Hub,Ehrlich Enterprise,Ride Base,Cleve Observatory"
+ },
+ {
+ "name": "63 Eridani",
+ "pos_x": 76.53125,
+ "pos_y": -84.9375,
+ "pos_z": -133.84375,
+ "stations": "Hartlib Terminal,Simonyi Dock,McCulley Orbital,Galois' Inheritance,Turzillo Point"
+ },
+ {
+ "name": "63 G. Capricorni",
+ "pos_x": -19.1875,
+ "pos_y": -44.8125,
+ "pos_z": 52.53125,
+ "stations": "Liwei Gateway,Skvortsov Orbital,Ferguson Hub,Hunziker Station,Hennepin Lab,Walz Terminal,Veach Enterprise,Watts Installation,Noguchi Ring,Seega Orbital,Kuipers Terminal,Crippen Terminal"
+ },
+ {
+ "name": "63 Geminorum",
+ "pos_x": 31.9375,
+ "pos_y": 34.09375,
+ "pos_z": -100.71875,
+ "stations": "Fairbairn Enterprise,Chu Hub"
+ },
+ {
+ "name": "63 Omicron-2 Cancri",
+ "pos_x": 66.46875,
+ "pos_y": 87.03125,
+ "pos_z": -103.125,
+ "stations": "Land Colony,Russell Dock,Potagos Gateway"
+ },
+ {
+ "name": "63 Tauri",
+ "pos_x": -2.375,
+ "pos_y": -60.5,
+ "pos_z": -151.09375,
+ "stations": "Taylor Horizons"
+ },
+ {
+ "name": "64 Arietis",
+ "pos_x": -57.09375,
+ "pos_y": -90.65625,
+ "pos_z": -178.34375,
+ "stations": "Weyn Dock,Boulle Landing,Rescue Ship - Weyn Dock"
+ },
+ {
+ "name": "64 Ceti",
+ "pos_x": -37.0625,
+ "pos_y": -100.0625,
+ "pos_z": -79.125,
+ "stations": "Jensen Gateway,Cochrane Depot"
+ },
+ {
+ "name": "64 G. Capricorni",
+ "pos_x": -77.84375,
+ "pos_y": -104.03125,
+ "pos_z": 123,
+ "stations": "Speke Point,Brackett Mine,Nielsen Relay"
+ },
+ {
+ "name": "64 Geminorum",
+ "pos_x": 32,
+ "pos_y": 62.375,
+ "pos_z": -161.6875,
+ "stations": "Fawcett Gateway"
+ },
+ {
+ "name": "64 Piscium",
+ "pos_x": -44.84375,
+ "pos_y": -54.71875,
+ "pos_z": -29.125,
+ "stations": "Blodgett Port,Evans Settlement,Miller's Inheritance,Godwin Installation,Barry Dock"
+ },
+ {
+ "name": "64 Tauri",
+ "pos_x": -3.5,
+ "pos_y": -58.53125,
+ "pos_z": -150.375,
+ "stations": "Sheffield Penal colony,Dummer Horizons"
+ },
+ {
+ "name": "65 Kappa Tauri",
+ "pos_x": -12.90625,
+ "pos_y": -47.3125,
+ "pos_z": -145.9375,
+ "stations": "Levchenko Terminal,Windt Relay"
+ },
+ {
+ "name": "66 Andromedae",
+ "pos_x": -120.4375,
+ "pos_y": -28.90625,
+ "pos_z": -136.59375,
+ "stations": "Wyeth's Folly"
+ },
+ {
+ "name": "66 Ceti",
+ "pos_x": -17.4375,
+ "pos_y": -109.78125,
+ "pos_z": -66.46875,
+ "stations": "Barnes Dock"
+ },
+ {
+ "name": "66 Draconis",
+ "pos_x": -164.71875,
+ "pos_y": 46.3125,
+ "pos_z": -16.0625,
+ "stations": "Forbes Port,Filter Dock"
+ },
+ {
+ "name": "67 Tauri",
+ "pos_x": -12.21875,
+ "pos_y": -45.625,
+ "pos_z": -140.34375,
+ "stations": "Fuchs Mines,Penn Settlement,Marley Silo"
+ },
+ {
+ "name": "68 Draconis",
+ "pos_x": -151.46875,
+ "pos_y": 41,
+ "pos_z": -15.96875,
+ "stations": "Potagos Arsenal,Arkwright Port,Powers Stop"
+ },
+ {
+ "name": "68 Eridani",
+ "pos_x": 32.09375,
+ "pos_y": -34.125,
+ "pos_z": -68.5625,
+ "stations": "Voss Dock,Gerst Orbital"
+ },
+ {
+ "name": "68 Upsilon Pegasi",
+ "pos_x": -137.1875,
+ "pos_y": -98.5625,
+ "pos_z": -22.5625,
+ "stations": "Allen Plant"
+ },
+ {
+ "name": "69 e Herculis",
+ "pos_x": -128.3125,
+ "pos_y": 97,
+ "pos_z": 70.0625,
+ "stations": "Jones Lab,Akers Station"
+ },
+ {
+ "name": "69 G. Carinae",
+ "pos_x": 50.78125,
+ "pos_y": -14.28125,
+ "pos_z": 3.15625,
+ "stations": "Lounge Port,Haipeng Port,Boswell Terminal,Adams' Progress,Alas Station,Eschbach Landing,Moore Base,Gardner Point,Marshburn's Progress,Velazquez Holdings"
+ },
+ {
+ "name": "69 Tau Ophiuchi",
+ "pos_x": -58,
+ "pos_y": 18.3125,
+ "pos_z": 156,
+ "stations": "Cowper Point"
+ },
+ {
+ "name": "69 Upsilon Tauri",
+ "pos_x": -13.59375,
+ "pos_y": -45.96875,
+ "pos_z": -146.125,
+ "stations": "Hodgson Dock,Stasheff Enterprise,Rolland Landing,Nachtigal Horizons,Kozlov Survey"
+ },
+ {
+ "name": "7 Alpha Lacertae",
+ "pos_x": -99.84375,
+ "pos_y": -11.71875,
+ "pos_z": -20.65625,
+ "stations": "Virchow Orbital,Rosenberg Prospect,Leclerc Hub,Garratt Hub,Dampier Depot"
+ },
+ {
+ "name": "7 Andromedae",
+ "pos_x": -75.34375,
+ "pos_y": -14.28125,
+ "pos_z": -23.53125,
+ "stations": "Papin City,Hardy Landing,Ross Observatory"
+ },
+ {
+ "name": "7 Canum Venaticorum",
+ "pos_x": -46.125,
+ "pos_y": 132.9375,
+ "pos_z": -38.90625,
+ "stations": "Brosnan Colony,Stith Terminal,Hinz Arsenal,Kingsmill Platform"
+ },
+ {
+ "name": "7 G. Aquilae",
+ "pos_x": -116.90625,
+ "pos_y": 2.53125,
+ "pos_z": 141.1875,
+ "stations": "White Platform,Francisco de Almeida Platform,Meredith's Inheritance"
+ },
+ {
+ "name": "7 Kappa Delphini",
+ "pos_x": -76.78125,
+ "pos_y": -31.78125,
+ "pos_z": 52.40625,
+ "stations": "Schottky Platform"
+ },
+ {
+ "name": "7 Pi-1 Orionis",
+ "pos_x": 18.40625,
+ "pos_y": -39.125,
+ "pos_z": -108,
+ "stations": "Melvin Dock,Quimby Orbital,Margulies Lab,Sarafanov Ring,Vernadsky Orbital,Faraday Ring,Huygens Enterprise,Grabe Ring,Garden Relay,McCandless Penal colony"
+ },
+ {
+ "name": "7 Zeta-2 Lyrae",
+ "pos_x": -136.90625,
+ "pos_y": 45.65625,
+ "pos_z": 58,
+ "stations": "Antonelli Station,Rand Silo,Fullerton Platform,Baudin Plant"
+ },
+ {
+ "name": "70 Ophiuchi",
+ "pos_x": -8.21875,
+ "pos_y": 3.09375,
+ "pos_z": 14.0625,
+ "stations": "Rushworth Hub,Luk Station,Ings Enterprise,Cowper Exchange,Tevis Terminal"
+ },
+ {
+ "name": "70 Tauri",
+ "pos_x": 0.40625,
+ "pos_y": -57.46875,
+ "pos_z": -142.71875,
+ "stations": "Knight Base,Gann Horizons"
+ },
+ {
+ "name": "70 Virginis",
+ "pos_x": 5.9375,
+ "pos_y": 56.25,
+ "pos_z": 15.46875,
+ "stations": "Fawcett Hub,Navigator's Inheritance,MacLean Terminal,Vasilyev Dock,Frobenius Base,Blair Installation,Converse Horizons,Scalzi Keep"
+ },
+ {
+ "name": "71 Epsilon Piscium",
+ "pos_x": -82,
+ "pos_y": -148.125,
+ "pos_z": -66.3125,
+ "stations": "Petaja Installation,Reis Orbital,Hermite Holdings"
+ },
+ {
+ "name": "71 Omicron Geminorum",
+ "pos_x": 13.96875,
+ "pos_y": 69.84375,
+ "pos_z": -150.3125,
+ "stations": "Binder Vision,Malaspina Platform,Ingstad Vision"
+ },
+ {
+ "name": "72 Herculis",
+ "pos_x": -32.875,
+ "pos_y": 24.6875,
+ "pos_z": 22.21875,
+ "stations": "Wang Ring"
+ },
+ {
+ "name": "72 Ophiuchi",
+ "pos_x": -50.65625,
+ "pos_y": 20.375,
+ "pos_z": 67.53125,
+ "stations": "Gerst Gateway,Humboldt Vision,Vernadsky City,Pierce Enterprise,Hartog Installation,May Installation"
+ },
+ {
+ "name": "73 Rho Cygni",
+ "pos_x": -123.1875,
+ "pos_y": -10,
+ "pos_z": -2.46875,
+ "stations": "Edgeworth Platform,Gurragchaa Hub,Schottky Survey"
+ },
+ {
+ "name": "74 G. Aquarii",
+ "pos_x": -109.8125,
+ "pos_y": -89.5,
+ "pos_z": 77.5,
+ "stations": "Van Scyoc Vision,Litke Refinery"
+ },
+ {
+ "name": "76 Sigma Ceti",
+ "pos_x": 7.71875,
+ "pos_y": -77.71875,
+ "pos_z": -38.53125,
+ "stations": "Aksyonov Orbital,Garay Station,Piper Market,Hennen Station,McCandless Orbital,Shaw Orbital,Avicenna Point,Hawkes Lab,Jiushao Base"
+ },
+ {
+ "name": "77 Aquarii",
+ "pos_x": -49.28125,
+ "pos_y": -117.90625,
+ "pos_z": 41.5,
+ "stations": "Chertok City,Bauschinger Hub,Pratchett Depot"
+ },
+ {
+ "name": "77 Kappa Geminorum",
+ "pos_x": 36.5,
+ "pos_y": 54.25,
+ "pos_z": -125.375,
+ "stations": "Elion Lab"
+ },
+ {
+ "name": "78 Piscium",
+ "pos_x": -92.15625,
+ "pos_y": -68.375,
+ "pos_z": -71.15625,
+ "stations": "Slusser Lab"
+ },
+ {
+ "name": "78 Ursae Majoris",
+ "pos_x": -35.03125,
+ "pos_y": 72.53125,
+ "pos_z": -19.9375,
+ "stations": "Seddon Gateway,Rominger Dock,Kizim Hub,Haignere Station,Teller Terminal,Townshend Hub,Stapledon Enterprise,Read Gateway,Berners-Lee City,Treshchov Gateway,Arrhenius Orbital,Kwolek Survey"
+ },
+ {
+ "name": "79 Ceti",
+ "pos_x": -7,
+ "pos_y": -104.34375,
+ "pos_z": -72.21875,
+ "stations": "Guidoni Station,Barbaro Relay,Thoreau Enterprise,Baker Installation"
+ },
+ {
+ "name": "79 Psi-2 Piscium",
+ "pos_x": -89.71875,
+ "pos_y": -102.53125,
+ "pos_z": -72.46875,
+ "stations": "Ackerman Base,Siegel Landing"
+ },
+ {
+ "name": "8 Alpha-1 Libra",
+ "pos_x": 19.46875,
+ "pos_y": 45.625,
+ "pos_z": 56.125,
+ "stations": "Hire Hub"
+ },
+ {
+ "name": "8 Gamma Coronae Borealis",
+ "pos_x": -60.53125,
+ "pos_y": 114.1875,
+ "pos_z": 68.03125,
+ "stations": "Neumann Terminal,Love Enterprise,Armstrong Ring,Bounds Vision,McCandless Hub"
+ },
+ {
+ "name": "8 Kappa Piscium",
+ "pos_x": -87.28125,
+ "pos_y": -126.0625,
+ "pos_z": 7.4375,
+ "stations": "Leestma Settlement,Sekowski Survey"
+ },
+ {
+ "name": "8 Piscis Austrini",
+ "pos_x": -50.875,
+ "pos_y": -139.9375,
+ "pos_z": 119.03125,
+ "stations": "Gehry Dock,Stabenow Prospect"
+ },
+ {
+ "name": "80 e Piscium",
+ "pos_x": -48.09375,
+ "pos_y": -97.84375,
+ "pos_z": -42.96875,
+ "stations": "Winne Gateway,Ehrlich Dock,Merle's Folly,Popper Works,Deluc Orbital,Nourse Camp,Shukor Penal colony,Ray Vista,Cockrell Prospect"
+ },
+ {
+ "name": "80 Leonis",
+ "pos_x": 91.6875,
+ "pos_y": 156.6875,
+ "pos_z": -17.5,
+ "stations": "Isherwood Dock,Baker Market,Stevin Installation"
+ },
+ {
+ "name": "81 Leonis",
+ "pos_x": 45.9375,
+ "pos_y": 132.625,
+ "pos_z": -28.46875,
+ "stations": "Guidoni Ring"
+ },
+ {
+ "name": "82 Eridani",
+ "pos_x": 10.40625,
+ "pos_y": -16.3125,
+ "pos_z": -3.75,
+ "stations": "Hackworth Settlement,Bresnik Port,Godwin Vision,Petaja Depot,Roosa Dock"
+ },
+ {
+ "name": "82 Pegasi",
+ "pos_x": -116.8125,
+ "pos_y": -138.53125,
+ "pos_z": -23.75,
+ "stations": "Darwin Orbital,Rzeppa Base,Grechko Dock,Jemison Terminal"
+ },
+ {
+ "name": "83 Aquarii",
+ "pos_x": -100.65625,
+ "pos_y": -178.625,
+ "pos_z": 42.96875,
+ "stations": "Wul Vision,Ayerdhal Installation"
+ },
+ {
+ "name": "83 Cancri",
+ "pos_x": 54.21875,
+ "pos_y": 86.8125,
+ "pos_z": -84.28125,
+ "stations": "Mallett Dock,Bergerac Gateway,Williams Hub,Sheckley Dock,Zamyatin Hub"
+ },
+ {
+ "name": "83 Tau Piscium",
+ "pos_x": -111.28125,
+ "pos_y": -90.125,
+ "pos_z": -89.46875,
+ "stations": "Miletus Beacon,Leiber Settlement,Bolger Plant,Snodgrass' Progress"
+ },
+ {
+ "name": "84 Ceti",
+ "pos_x": -5.5625,
+ "pos_y": -58.15625,
+ "pos_z": -44.9375,
+ "stations": "Dozois Market,Boucher Terminal"
+ },
+ {
+ "name": "85 Pegasi",
+ "pos_x": -30.75,
+ "pos_y": -22.40625,
+ "pos_z": -11.40625,
+ "stations": "Carver Survey,Borman Terminal,Buckland Gateway,Sturckow Installation"
+ },
+ {
+ "name": "86 Aquarii",
+ "pos_x": -50.65625,
+ "pos_y": -198.625,
+ "pos_z": 69.03125,
+ "stations": "Milne Mine,Beer Terminal,Shimizu Base,Anderson Arsenal"
+ },
+ {
+ "name": "86 Mu Herculis",
+ "pos_x": -19.5,
+ "pos_y": 11.53125,
+ "pos_z": 14.875,
+ "stations": "Gauss Platform,Kanwar Orbital,Scheutz Hub,Cole Oasis"
+ },
+ {
+ "name": "87 Mu Ceti",
+ "pos_x": -17.09375,
+ "pos_y": -57.5,
+ "pos_z": -58.9375,
+ "stations": "Benford's Inheritance,Dobrovolski Platform,Gell-Mann Port"
+ },
+ {
+ "name": "88 d Tauri",
+ "pos_x": 16.1875,
+ "pos_y": -60.0625,
+ "pos_z": -137,
+ "stations": "la Cosa Landing,Barbosa Prospect,Hand Beacon"
+ },
+ {
+ "name": "89 Centauri",
+ "pos_x": 126.84375,
+ "pos_y": 57.96875,
+ "pos_z": 55.03125,
+ "stations": "Kennicott Platform"
+ },
+ {
+ "name": "89 Leonis",
+ "pos_x": 44.34375,
+ "pos_y": 76.75,
+ "pos_z": -5.03125,
+ "stations": "Powers Landing,Binet Port,Sanger Dock,Altman's Progress,Horch Survey,Rozhdestvensky Base,de Balboa Settlement"
+ },
+ {
+ "name": "9 Aurigae",
+ "pos_x": -32.75,
+ "pos_y": 10.5,
+ "pos_z": -78.5625,
+ "stations": "Zahn Station,Lehtonen Orbital,Rubruck Station,Cochrane Base,Kennicott Enterprise,Kummer Hub,Mohun's Inheritance,Tarski Point,Hill Orbital,Fancher Orbital,Niven's Inheritance,McMullen Beacon,Hunt Enterprise,Blaschke Hub"
+ },
+ {
+ "name": "9 Ceti",
+ "pos_x": -18.9375,
+ "pos_y": -65.3125,
+ "pos_z": -3.28125,
+ "stations": "Fanning Vision,Payson Bastion"
+ },
+ {
+ "name": "9 Comae Berenices",
+ "pos_x": 8.46875,
+ "pos_y": 176.46875,
+ "pos_z": -18.46875,
+ "stations": "Springer Vision"
+ },
+ {
+ "name": "9 G. Carinae",
+ "pos_x": 108.65625,
+ "pos_y": -48.65625,
+ "pos_z": -17.90625,
+ "stations": "Ansari Hangar"
+ },
+ {
+ "name": "9 Iota Piscis Austrini",
+ "pos_x": -30.125,
+ "pos_y": -156.8125,
+ "pos_z": 127.34375,
+ "stations": "Binney Colony"
+ },
+ {
+ "name": "9 Lacertae",
+ "pos_x": -166.28125,
+ "pos_y": -17.71875,
+ "pos_z": -38.84375,
+ "stations": "Wallace Vision,Alexandria Arsenal"
+ },
+ {
+ "name": "9 Omicron Virginis",
+ "pos_x": 59.375,
+ "pos_y": 152.03125,
+ "pos_z": 2,
+ "stations": "Morris Survey,Schmidt Enterprise"
+ },
+ {
+ "name": "9 Puppis",
+ "pos_x": 42.46875,
+ "pos_y": 6.59375,
+ "pos_z": -32.40625,
+ "stations": "Markov Asylum,Fuglesang Horizons"
+ },
+ {
+ "name": "9 Ursae Minoris",
+ "pos_x": -77.625,
+ "pos_y": 74.5,
+ "pos_z": -27.90625,
+ "stations": "Noli Point,Hartog Settlement,Grothendieck Relay"
+ },
+ {
+ "name": "90 G. Canis Majoris",
+ "pos_x": 71,
+ "pos_y": -17.28125,
+ "pos_z": -42.34375,
+ "stations": "Reilly Terminal,Howard Platform,Miller Reach"
+ },
+ {
+ "name": "91 Sigma-1 Tauri",
+ "pos_x": 5.875,
+ "pos_y": -49.03125,
+ "pos_z": -138.53125,
+ "stations": "Schwann Works,Haller Depot,Martin's Folly"
+ },
+ {
+ "name": "93 Rho Piscium",
+ "pos_x": -42.875,
+ "pos_y": -55.65625,
+ "pos_z": -42.84375,
+ "stations": "Brash City,Hume's Claim"
+ },
+ {
+ "name": "95 Tauri",
+ "pos_x": -10,
+ "pos_y": -37,
+ "pos_z": -152.6875,
+ "stations": "Svavarsson Beacon,Nansen Silo"
+ },
+ {
+ "name": "97 i Tauri",
+ "pos_x": 4.875,
+ "pos_y": -44.15625,
+ "pos_z": -161.1875,
+ "stations": "Converse Ring,Goonan Arsenal"
+ },
+ {
+ "name": "98 Aquarii",
+ "pos_x": -43.9375,
+ "pos_y": -152.625,
+ "pos_z": 38.53125,
+ "stations": "McKee Terminal,Stross Installation,Hornoch Enterprise"
+ },
+ {
+ "name": "Aakuagsakh",
+ "pos_x": -47,
+ "pos_y": 43.59375,
+ "pos_z": 169.375,
+ "stations": "Bombelli Holdings,Abe Point,Smith Vision"
+ },
+ {
+ "name": "Aakuman",
+ "pos_x": 63.875,
+ "pos_y": 161.5625,
+ "pos_z": -22.875,
+ "stations": "al-Khowarizmi Outpost,Cramer Penal colony,Perga's Claim"
+ },
+ {
+ "name": "Aakushu",
+ "pos_x": 73.9375,
+ "pos_y": -80.84375,
+ "pos_z": 116.625,
+ "stations": "Flagg Dock,Hawker Depot"
+ },
+ {
+ "name": "Aapelinja",
+ "pos_x": 17.09375,
+ "pos_y": 139.84375,
+ "pos_z": -7.0625,
+ "stations": "Treshchov Gateway,Voss City,Jensen Terminal,Burbank Gateway,Crown Gateway,Hennepin Market,Weinbaum Colony,Lazarev Penal colony"
+ },
+ {
+ "name": "Aapep",
+ "pos_x": 80.96875,
+ "pos_y": 18.9375,
+ "pos_z": -163.625,
+ "stations": "Tavernier Horizons,Holub Terminal,Dedman Terminal"
+ },
+ {
+ "name": "Aarti",
+ "pos_x": 163.15625,
+ "pos_y": -64.53125,
+ "pos_z": -10.6875,
+ "stations": "Marius Station,Gonnessiat Landing,Narbeth Survey,Bothezat Survey"
+ },
+ {
+ "name": "Aasgaa",
+ "pos_x": 97.3125,
+ "pos_y": 44.15625,
+ "pos_z": 57.9375,
+ "stations": "Steiner Platform,Obruchev Settlement,Watts Enterprise,Powell Prospect"
+ },
+ {
+ "name": "Aasgananu",
+ "pos_x": -38.8125,
+ "pos_y": -62.90625,
+ "pos_z": -73.875,
+ "stations": "Houssay Ring,Sturckow Platform,Nicolet Works,Lovelace City,McQuay Silo"
+ },
+ {
+ "name": "Aasgay",
+ "pos_x": 81.28125,
+ "pos_y": 9.21875,
+ "pos_z": 27.40625,
+ "stations": "Berezin Town,Higginbotham Station,Solovyev Hub,Drebbel Hub,Glidden Palace"
+ },
+ {
+ "name": "AB Pictoris",
+ "pos_x": 133.875,
+ "pos_y": -67.84375,
+ "pos_z": -7.0625,
+ "stations": "Mohr City"
+ },
+ {
+ "name": "Abaana",
+ "pos_x": -16.28125,
+ "pos_y": -109.75,
+ "pos_z": 24.09375,
+ "stations": "Edwards Installation,Deutsch Installation"
+ },
+ {
+ "name": "Abanda",
+ "pos_x": 83.3125,
+ "pos_y": -229.875,
+ "pos_z": 51.90625,
+ "stations": "Deutsch Mine"
+ },
+ {
+ "name": "Abasina",
+ "pos_x": 84.65625,
+ "pos_y": -85.0625,
+ "pos_z": 174,
+ "stations": "Truman Terminal,Riebe Survey,Friesner Depot,Vasilyev Installation"
+ },
+ {
+ "name": "Abasses",
+ "pos_x": -34.1875,
+ "pos_y": -102.375,
+ "pos_z": 81,
+ "stations": "Shimizu Orbital,Morrow Vision,Hamilton Point"
+ },
+ {
+ "name": "Abassong",
+ "pos_x": -14.5,
+ "pos_y": -114.40625,
+ "pos_z": -127.53125,
+ "stations": "Acaba Settlement,Hedley Terminal"
+ },
+ {
+ "name": "Abast Samo",
+ "pos_x": -44.25,
+ "pos_y": -122.28125,
+ "pos_z": -24.4375,
+ "stations": "Kaku Horizons,Boe Orbital"
+ },
+ {
+ "name": "Abazahua",
+ "pos_x": 109.78125,
+ "pos_y": -3.90625,
+ "pos_z": -103.75,
+ "stations": "Vinge's Inheritance,Mohun Survey"
+ },
+ {
+ "name": "Abazates",
+ "pos_x": 160.0625,
+ "pos_y": -32.90625,
+ "pos_z": -12.71875,
+ "stations": "Wood Base,Ryazanski Platform"
+ },
+ {
+ "name": "Abazi",
+ "pos_x": -71.1875,
+ "pos_y": 26.4375,
+ "pos_z": 43.875,
+ "stations": "Messerschmid Enterprise,Ozanne Landing"
+ },
+ {
+ "name": "Abe",
+ "pos_x": -30.125,
+ "pos_y": -160.875,
+ "pos_z": 19.40625,
+ "stations": "Davies Penal colony,Johnson Port"
+ },
+ {
+ "name": "Abi",
+ "pos_x": -32.34375,
+ "pos_y": -29.90625,
+ "pos_z": 10.625,
+ "stations": "Grechko Dock,Sommerfeld Orbital,Ferguson Terminal"
+ },
+ {
+ "name": "Abibitoa",
+ "pos_x": -120.78125,
+ "pos_y": 8.53125,
+ "pos_z": 61.8125,
+ "stations": "Andree Plant"
+ },
+ {
+ "name": "Abidii",
+ "pos_x": 30.15625,
+ "pos_y": -73.71875,
+ "pos_z": -131.40625,
+ "stations": "King Reach"
+ },
+ {
+ "name": "Abidji",
+ "pos_x": -147.28125,
+ "pos_y": 55,
+ "pos_z": 69.53125,
+ "stations": "Barreiros Platform,Beliaev Hub,Nehsi Landing"
+ },
+ {
+ "name": "Abikabiates",
+ "pos_x": 59.34375,
+ "pos_y": -151.4375,
+ "pos_z": -54.625,
+ "stations": "Brashear Prospect,Laird Beacon,Anderson Prospect"
+ },
+ {
+ "name": "Abikudi",
+ "pos_x": 4.9375,
+ "pos_y": -140.625,
+ "pos_z": 117.375,
+ "stations": "Hencke Orbital,Horrocks Survey,de Andrade Hub"
+ },
+ {
+ "name": "Abikuolu",
+ "pos_x": 173.40625,
+ "pos_y": -139.625,
+ "pos_z": 63.4375,
+ "stations": "Pearse Base"
+ },
+ {
+ "name": "Abora",
+ "pos_x": 18.4375,
+ "pos_y": -117.84375,
+ "pos_z": 128.75,
+ "stations": "Shirazi Vision,Gustav Sporer Prospect,Csoma Penal colony"
+ },
+ {
+ "name": "Abormotwa",
+ "pos_x": 109.1875,
+ "pos_y": 77,
+ "pos_z": -34.5,
+ "stations": "Apt Ring"
+ },
+ {
+ "name": "Aborovi",
+ "pos_x": -123.71875,
+ "pos_y": -19.0625,
+ "pos_z": 89.1875,
+ "stations": "Clauss Reach,Doyle Asylum"
+ },
+ {
+ "name": "Abreda",
+ "pos_x": 117.15625,
+ "pos_y": -200.59375,
+ "pos_z": 61.1875,
+ "stations": "Flammarion Orbital,Tully Vision,Steakley Reach"
+ },
+ {
+ "name": "Abres",
+ "pos_x": -64.25,
+ "pos_y": 76.0625,
+ "pos_z": -67.65625,
+ "stations": "Russell Hub,Pangborn Dock,Eisenstein Dock,Cobb's Pride,Tanaka Plant,Antonio de Andrade Works"
+ },
+ {
+ "name": "Abriama",
+ "pos_x": -61.5,
+ "pos_y": 122.3125,
+ "pos_z": -95.5,
+ "stations": "Davis City,Napier Colony,Simak's Folly,Andrews Depot"
+ },
+ {
+ "name": "Abrigi",
+ "pos_x": 190.875,
+ "pos_y": -181.84375,
+ "pos_z": 25.625,
+ "stations": "Marth Stop,Goldreich Relay"
+ },
+ {
+ "name": "Abriguaymir",
+ "pos_x": 104,
+ "pos_y": 42.46875,
+ "pos_z": -93.6875,
+ "stations": "Babcock Base,Sullivan Settlement,Czerny Settlement"
+ },
+ {
+ "name": "Abrocmii",
+ "pos_x": -13.4375,
+ "pos_y": -61.59375,
+ "pos_z": -67.03125,
+ "stations": "Rennie City,Ramsbottom Station,Ray Station,Shepard Gateway,Johnson Gateway,Knapp Dock,Ham Ring,Patrick Station,Nespoli Prospect,Morin Colony,Garn Station,Coblentz Colony"
+ },
+ {
+ "name": "Abrog",
+ "pos_x": -124.15625,
+ "pos_y": -42.03125,
+ "pos_z": 7.28125,
+ "stations": "Thagard Ring,Velazquez Vision,Wolf Platform,Gunn Depot,Fermi City"
+ },
+ {
+ "name": "Abrogo",
+ "pos_x": 20.8125,
+ "pos_y": 16.75,
+ "pos_z": -13.3125,
+ "stations": "Haller Installation"
+ },
+ {
+ "name": "Abroin",
+ "pos_x": -94.53125,
+ "pos_y": 110.09375,
+ "pos_z": -40.5,
+ "stations": "Campbell Horizons,Sheckley Settlement,Galois Colony,Carleson Terminal"
+ },
+ {
+ "name": "Abuk",
+ "pos_x": 60.34375,
+ "pos_y": -109.9375,
+ "pos_z": 132.53125,
+ "stations": "Sugano Hub,Neugebauer Dock,Hill Port,Shepherd Terminal"
+ },
+ {
+ "name": "Abukunin",
+ "pos_x": -69.3125,
+ "pos_y": -7.4375,
+ "pos_z": 61.9375,
+ "stations": "Janifer Camp,Reilly Hub,Titov Installation"
+ },
+ {
+ "name": "Abukunn",
+ "pos_x": 81.1875,
+ "pos_y": -142.25,
+ "pos_z": 119.46875,
+ "stations": "Helffrich Outpost"
+ },
+ {
+ "name": "Aburaces",
+ "pos_x": 42.625,
+ "pos_y": -141.875,
+ "pos_z": 162.78125,
+ "stations": "Dayuan's Folly,Allen St. John Terminal,von Zach Terminal"
+ },
+ {
+ "name": "Aburokul",
+ "pos_x": -117.40625,
+ "pos_y": 99.75,
+ "pos_z": -54.40625,
+ "stations": "al-Din Station"
+ },
+ {
+ "name": "Aburu",
+ "pos_x": -6.46875,
+ "pos_y": -34.59375,
+ "pos_z": 89.59375,
+ "stations": "Bykovsky Refinery,Benford Works,Dyomin Plant,Bethe Landing"
+ },
+ {
+ "name": "Aburukan",
+ "pos_x": 71,
+ "pos_y": -176.53125,
+ "pos_z": 143.40625,
+ "stations": "Dhawan Station,Brothers Station"
+ },
+ {
+ "name": "AC +26 37030",
+ "pos_x": -29.6875,
+ "pos_y": 65.21875,
+ "pos_z": 35.6875,
+ "stations": "Culbertson Port,Blair Installation,Parry Hub,Kimbrough Settlement"
+ },
+ {
+ "name": "AC +40 428-42",
+ "pos_x": 1.875,
+ "pos_y": 76.96875,
+ "pos_z": -51.875,
+ "stations": "Shinn Station,Friend's Progress,Stevens' Folly"
+ },
+ {
+ "name": "AC +67 1894",
+ "pos_x": -41.125,
+ "pos_y": 29.28125,
+ "pos_z": -61.8125,
+ "stations": "Glenn Point,Blodgett Colony,Bell Penitentiary"
+ },
+ {
+ "name": "Ac Yai",
+ "pos_x": 137.71875,
+ "pos_y": -59.09375,
+ "pos_z": 40.625,
+ "stations": "August von Steinheil Dock,Porco Arsenal"
+ },
+ {
+ "name": "Ac Yama",
+ "pos_x": 127.78125,
+ "pos_y": -32.1875,
+ "pos_z": -51.28125,
+ "stations": "Archer Bastion,Kornbluth Orbital,Neff Enterprise"
+ },
+ {
+ "name": "Ac Yaming",
+ "pos_x": 133.4375,
+ "pos_y": -200.375,
+ "pos_z": 131.875,
+ "stations": "Gabrielli Relay,Barcelo Silo"
+ },
+ {
+ "name": "Ac Yanto",
+ "pos_x": 83.09375,
+ "pos_y": 35.9375,
+ "pos_z": 98.15625,
+ "stations": "Staden Beacon,Boucher Installation,Przhevalsky Freeport"
+ },
+ {
+ "name": "Ac Yanyamam",
+ "pos_x": -21.4375,
+ "pos_y": 27.84375,
+ "pos_z": -127.875,
+ "stations": "Isherwood Survey,Jordan Holdings"
+ },
+ {
+ "name": "Ac Yariu",
+ "pos_x": 83.71875,
+ "pos_y": -77.03125,
+ "pos_z": 7.75,
+ "stations": "Lambert City,MacCready Hub"
+ },
+ {
+ "name": "Ac Yax",
+ "pos_x": 2.34375,
+ "pos_y": 83.625,
+ "pos_z": 138.21875,
+ "stations": "Clervoy Settlement"
+ },
+ {
+ "name": "Ac Yax Baru",
+ "pos_x": 103.71875,
+ "pos_y": -0.65625,
+ "pos_z": 99.0625,
+ "stations": "Leibniz Dock,Pangborn Mine,Garan Port,Walz Dock,Hawking Terminal,Grabe Port,Cavalieri Camp"
+ },
+ {
+ "name": "Acamar",
+ "pos_x": 73.375,
+ "pos_y": -140.34375,
+ "pos_z": -30.78125,
+ "stations": "i Sola Platform,Yamamoto Hub,Abel Colony,Bigourdan Vision"
+ },
+ {
+ "name": "Acan",
+ "pos_x": -13.21875,
+ "pos_y": 88.5625,
+ "pos_z": 24.96875,
+ "stations": "King Dock,Rochon Legacy,Phillifent Dock,Marques Platform"
+ },
+ {
+ "name": "Acandizel",
+ "pos_x": 112.3125,
+ "pos_y": 74.9375,
+ "pos_z": -6.84375,
+ "stations": "Hammond Depot,Smith Prospect"
+ },
+ {
+ "name": "Acane",
+ "pos_x": 101.375,
+ "pos_y": 88.96875,
+ "pos_z": 76.6875,
+ "stations": "Sommerfeld Enterprise,Hornby Dock"
+ },
+ {
+ "name": "Acani",
+ "pos_x": 189.90625,
+ "pos_y": -173.96875,
+ "pos_z": 9.96875,
+ "stations": "Tayler Penal colony,Bartolomeu de Gusmao Vision,Howe Landing,Gunter Orbital"
+ },
+ {
+ "name": "Acantha",
+ "pos_x": -59.96875,
+ "pos_y": 99.21875,
+ "pos_z": 99.5625,
+ "stations": "MacVicar Settlement,Lopez de Legazpi Settlement,Laird Horizons"
+ },
+ {
+ "name": "Acanthu",
+ "pos_x": 117.125,
+ "pos_y": 38.46875,
+ "pos_z": 58.3125,
+ "stations": "Kadenyuk Survey,Noriega Landing"
+ },
+ {
+ "name": "Acants",
+ "pos_x": -98.96875,
+ "pos_y": -48.1875,
+ "pos_z": -90.21875,
+ "stations": "Bykovsky Station,Landsteiner Station,Gorbatko Terminal,Lovelace Terminal,Banks Hub,Ivins Enterprise,Hoard Gateway,Atiyah Base,Atiyah Penal colony,Moore Base"
+ },
+ {
+ "name": "Acatesano",
+ "pos_x": -39.96875,
+ "pos_y": -55.84375,
+ "pos_z": 97.3125,
+ "stations": "Tarelkin Base,Hughes-Fulford Stop"
+ },
+ {
+ "name": "Acawar",
+ "pos_x": -62.59375,
+ "pos_y": -63.625,
+ "pos_z": -159.6875,
+ "stations": "Ferguson Escape"
+ },
+ {
+ "name": "Achali",
+ "pos_x": 50.34375,
+ "pos_y": 27.4375,
+ "pos_z": 112.28125,
+ "stations": "Garratt Ring,Vinge Silo"
+ },
+ {
+ "name": "Achan",
+ "pos_x": 85.625,
+ "pos_y": -142.4375,
+ "pos_z": 128.6875,
+ "stations": "Lunney Stop,Harvey-Smith Arsenal"
+ },
+ {
+ "name": "Achansa",
+ "pos_x": 53.1875,
+ "pos_y": 24.25,
+ "pos_z": -107.71875,
+ "stations": "Patterson Installation,Lunan Terminal,Gunn Gateway,Camarda Arena"
+ },
+ {
+ "name": "Achapsugs",
+ "pos_x": 75.90625,
+ "pos_y": -151.84375,
+ "pos_z": -54.71875,
+ "stations": "Kerimov Hub,Krylov Landing,Ziewe Station"
+ },
+ {
+ "name": "Achar",
+ "pos_x": -41.25,
+ "pos_y": 92.6875,
+ "pos_z": -91.5625,
+ "stations": "Born City"
+ },
+ {
+ "name": "Ache",
+ "pos_x": -140.1875,
+ "pos_y": 41.46875,
+ "pos_z": -54.5625,
+ "stations": "Tall Oasis,Carpini Prospect"
+ },
+ {
+ "name": "Achehoongat",
+ "pos_x": 93.15625,
+ "pos_y": -133,
+ "pos_z": 102.65625,
+ "stations": "Dalgarno Station,Nomura Port,Baily Dock,Lavochkin Station,Fallows Vision,Tank Orbital,Okorafor Installation,Froud Port,Boss Hub,Grimwood Beacon,Humphreys' Progress,Burgess Prospect,van Houten Arsenal,MacGill Reach"
+ },
+ {
+ "name": "Achelous",
+ "pos_x": -76.9375,
+ "pos_y": 3.53125,
+ "pos_z": 19.96875,
+ "stations": "Mendel Hub,Thompson Gateway,Hennen Enterprise,Copernicus Dock,Rochon Vision,Marshall Survey,Okorafor Depot,Noon Landing,Bridger's Inheritance"
+ },
+ {
+ "name": "Achenar",
+ "pos_x": 67.5,
+ "pos_y": -119.46875,
+ "pos_z": 24.84375,
+ "stations": "Fortune's Loss,Dawes Hub,Macmillan Terminal,Jones Dock,Baker Terminal,Bell Terminal,Greenstein Silo,Wilson Vision,Baynes Horizons,Peters City,Kagawa Station"
+ },
+ {
+ "name": "Achian",
+ "pos_x": -154.8125,
+ "pos_y": -3.28125,
+ "pos_z": 30.4375,
+ "stations": "McKee Terminal"
+ },
+ {
+ "name": "Achini",
+ "pos_x": 136.4375,
+ "pos_y": -82.375,
+ "pos_z": -34.75,
+ "stations": "Moorcock Works,Hill Tinsley Colony,Alcock Platform,Ocampo Mines,Paul Station"
+ },
+ {
+ "name": "Achlys",
+ "pos_x": 1.1875,
+ "pos_y": -26.21875,
+ "pos_z": -71,
+ "stations": "Wallace Platform"
+ },
+ {
+ "name": "Achosi",
+ "pos_x": 2.25,
+ "pos_y": -29.25,
+ "pos_z": 138.3125,
+ "stations": "Margulis Orbital,Metz Camp"
+ },
+ {
+ "name": "Achrende",
+ "pos_x": 50.90625,
+ "pos_y": -134.46875,
+ "pos_z": -67.5,
+ "stations": "Vogel Enterprise,Vogel Hub,Resnik Orbital,Glushko Holdings,Rucker Hub"
+ },
+ {
+ "name": "Achreni",
+ "pos_x": 123.75,
+ "pos_y": -68.09375,
+ "pos_z": 47.3125,
+ "stations": "Jeans Colony,Fraknoi Installation,van de Hulst Station"
+ },
+ {
+ "name": "Achuar",
+ "pos_x": 26.09375,
+ "pos_y": -231.4375,
+ "pos_z": 5.9375,
+ "stations": "Mouchez Platform"
+ },
+ {
+ "name": "Acihaut",
+ "pos_x": -18.5,
+ "pos_y": 25.28125,
+ "pos_z": -4,
+ "stations": "Mastracchio Base,Payton Terminal,Gromov Town,Habermann Sanctuary"
+ },
+ {
+ "name": "Acionna",
+ "pos_x": 47.78125,
+ "pos_y": 16.90625,
+ "pos_z": 57.78125,
+ "stations": "Vasyutin Port,Carrier Mine"
+ },
+ {
+ "name": "Ackwada",
+ "pos_x": 42.78125,
+ "pos_y": -65.3125,
+ "pos_z": -11.34375,
+ "stations": "Birminghamport,Rush's Camp,Carter Hanger,MacVicar Port,Bokeili Colony,Barbaro Town,Smith Vision,George's Inheritance,Csoma Vision"
+ },
+ {
+ "name": "Ackycha",
+ "pos_x": -29.03125,
+ "pos_y": 39.8125,
+ "pos_z": -25.03125,
+ "stations": "Curie City"
+ },
+ {
+ "name": "Acokwa",
+ "pos_x": -52.59375,
+ "pos_y": -2.90625,
+ "pos_z": -92.3125,
+ "stations": "Dezhurov Hangar"
+ },
+ {
+ "name": "Acokwang",
+ "pos_x": 80.6875,
+ "pos_y": -111.0625,
+ "pos_z": 4.71875,
+ "stations": "Moore Platform,Zahn Colony"
+ },
+ {
+ "name": "Acokwech",
+ "pos_x": 106.9375,
+ "pos_y": 4.875,
+ "pos_z": 5.75,
+ "stations": "Dobrovolski Port,Grandin City"
+ },
+ {
+ "name": "Acokwus",
+ "pos_x": 116.5625,
+ "pos_y": -1.8125,
+ "pos_z": -130.4375,
+ "stations": "Franklin Colony,Dana Landing,Rochon Barracks"
+ },
+ {
+ "name": "Acoman",
+ "pos_x": 126.03125,
+ "pos_y": -32.3125,
+ "pos_z": -53.96875,
+ "stations": "Kinsey Outpost"
+ },
+ {
+ "name": "Acomatshi",
+ "pos_x": 113.21875,
+ "pos_y": 9.15625,
+ "pos_z": -130.59375,
+ "stations": "Volynov Point"
+ },
+ {
+ "name": "Acuruncegi",
+ "pos_x": -60.71875,
+ "pos_y": 117.625,
+ "pos_z": -123.46875,
+ "stations": "Bryusov Asylum"
+ },
+ {
+ "name": "Acurus",
+ "pos_x": -142.46875,
+ "pos_y": 10.40625,
+ "pos_z": 102.65625,
+ "stations": "Amnuel Terminal,Matthews Base,Marusek Colony,Thompson Enterprise,Friesner Orbital,Evans Point"
+ },
+ {
+ "name": "Adach",
+ "pos_x": -117.71875,
+ "pos_y": -85.125,
+ "pos_z": 82.4375,
+ "stations": "Bartoe Vision"
+ },
+ {
+ "name": "Adachit",
+ "pos_x": 107.28125,
+ "pos_y": -163.84375,
+ "pos_z": 44.3125,
+ "stations": "Wright Gateway,Hale Dock,Wang Vision,Bliss Port"
+ },
+ {
+ "name": "Adad",
+ "pos_x": -25.5625,
+ "pos_y": -56,
+ "pos_z": 10.15625,
+ "stations": "Alvares Terminal,Cook Hub,McAuley City,Banach Orbital,Archer Point,Morrison Terminal,Carrasco Station,Gwynn Station,Napier Orbital,Asimov Dock,Whitney Beacon"
+ },
+ {
+ "name": "Adan",
+ "pos_x": 116.5625,
+ "pos_y": -100.5625,
+ "pos_z": 0.5625,
+ "stations": "Bottego Arsenal,Ptack Dock"
+ },
+ {
+ "name": "Addo",
+ "pos_x": -10.125,
+ "pos_y": -81.75,
+ "pos_z": 40,
+ "stations": "Ferguson Refinery,Elcano Port,Jernigan Beacon"
+ },
+ {
+ "name": "Addovices",
+ "pos_x": -33.4375,
+ "pos_y": -40.65625,
+ "pos_z": -43.8125,
+ "stations": "Nakaya Vision,Onufrienko Base"
+ },
+ {
+ "name": "Addovichs",
+ "pos_x": -19.46875,
+ "pos_y": -168.375,
+ "pos_z": -18.34375,
+ "stations": "Aoki Port"
+ },
+ {
+ "name": "Addovii",
+ "pos_x": 59.84375,
+ "pos_y": 104.34375,
+ "pos_z": -81.09375,
+ "stations": "Novitski Horizons,Wegener Horizons,Galvani Hub"
+ },
+ {
+ "name": "Addovik",
+ "pos_x": 136.5625,
+ "pos_y": -60.875,
+ "pos_z": 5.4375,
+ "stations": "Lambert Orbital,Ziegler Dock,Blish Holdings,Nojiri Orbital,Goryu Survey"
+ },
+ {
+ "name": "Addovika",
+ "pos_x": 65.6875,
+ "pos_y": -181.15625,
+ "pos_z": 127.46875,
+ "stations": "Parker Vision,Fedden Dock,Jackson Platform,Roskam Survey"
+ },
+ {
+ "name": "Adeka",
+ "pos_x": 18.78125,
+ "pos_y": 116.9375,
+ "pos_z": 76.46875,
+ "stations": "Helms Orbital,Moresby Enterprise,Grissom Platform,Haberlandt Enterprise"
+ },
+ {
+ "name": "Adekalta",
+ "pos_x": 107.53125,
+ "pos_y": 99.59375,
+ "pos_z": -51.59375,
+ "stations": "Conklin Platform,Block Settlement,Longyear Terminal"
+ },
+ {
+ "name": "Adenates",
+ "pos_x": -51.4375,
+ "pos_y": -70.75,
+ "pos_z": 60.59375,
+ "stations": "Bailey Landing,Volterra Depot,Newcomb Landing"
+ },
+ {
+ "name": "Adenets",
+ "pos_x": -40.125,
+ "pos_y": 65.96875,
+ "pos_z": -35.5625,
+ "stations": "Bushkov Dock,Poincare Gateway,Kennicott Enterprise,Sabine Gateway,Vasquez de Coronado's Claim,Sarmiento de Gamboa Depot,Wilkes Gateway,Martin Gateway,Henslow Market,Moran Legacy,Dummer Port,Napier Enterprise"
+ },
+ {
+ "name": "Adenses",
+ "pos_x": 19.84375,
+ "pos_y": 64.75,
+ "pos_z": -59.6875,
+ "stations": "Leiber Dock,Quimper Dock,Palmer Mines"
+ },
+ {
+ "name": "Adensu",
+ "pos_x": -94.84375,
+ "pos_y": -75.09375,
+ "pos_z": -94.0625,
+ "stations": "Barreiros Horizons,Bradley Plant"
+ },
+ {
+ "name": "Adeo",
+ "pos_x": -81.625,
+ "pos_y": 31.40625,
+ "pos_z": 27.1875,
+ "stations": "Foda Port,Vinci Hub,Cormack Dock,Dobrovolski City,Ramelli Dock,Stevin Beacon,Yang Orbital,Bohr Station,Pribylov Terminal,Sverdrup Enterprise,Ivanishin Station"
+ },
+ {
+ "name": "Adepti",
+ "pos_x": -74.09375,
+ "pos_y": 36.5625,
+ "pos_z": -40.1875,
+ "stations": "Iqbal Dock,Sargent Survey,Lee Enterprise"
+ },
+ {
+ "name": "Adils",
+ "pos_x": -61.1875,
+ "pos_y": -28.53125,
+ "pos_z": -80.4375,
+ "stations": "Marconi Port,Comino Installation,Keyes Horizons"
+ },
+ {
+ "name": "Adit",
+ "pos_x": 174.0625,
+ "pos_y": -100.28125,
+ "pos_z": 126.0625,
+ "stations": "Auwers Terminal,Lewis Port,Pellegrino Penal colony,Schroter Station"
+ },
+ {
+ "name": "Aditi",
+ "pos_x": 72.875,
+ "pos_y": -337.84375,
+ "pos_z": -159.40625,
+ "stations": "SMJL Central,PEW-1901,Cremona Arsenal,Beaufoy Works,Fabian Enterprise"
+ },
+ {
+ "name": "Aditjana",
+ "pos_x": -73.4375,
+ "pos_y": -134.8125,
+ "pos_z": 75.15625,
+ "stations": "Escobar Platform"
+ },
+ {
+ "name": "Aditjargl",
+ "pos_x": -0.53125,
+ "pos_y": 66.75,
+ "pos_z": 86.375,
+ "stations": "Wolf Ring,Garneau Station,Gidzenko Dock,Satcher Hub,Trinh Station,Nearchus Prospect,Rontgen Enterprise,Watson Enterprise,Rond d'Alembert Holdings,Worlidge Station,Scheutz Orbital,Horch Works,DeLucas Station,Soddy's Progress,Rocklynne's Progress"
+ },
+ {
+ "name": "Aditjari",
+ "pos_x": 85.90625,
+ "pos_y": -103.125,
+ "pos_z": 155.21875,
+ "stations": "Barnard Hub,Araki Vision,Marriott Enterprise,Bharadwaj Keep,Toll's Progress"
+ },
+ {
+ "name": "Adityan",
+ "pos_x": -80.875,
+ "pos_y": -68.96875,
+ "pos_z": 23.03125,
+ "stations": "Leavitt Hub,Kooi Station,Ramanujan Hub,Kafka Oasis,Holland Horizons,Konscak Penal colony,Engle Hub,Hopkins City,Huygens Enterprise,Diesel Enterprise,Fearn Settlement,Fullerton Station,Allen Market,Margulis Station"
+ },
+ {
+ "name": "Adiva",
+ "pos_x": 102.09375,
+ "pos_y": 2.875,
+ "pos_z": 98.40625,
+ "stations": "Bosch Survey,Hunt Beacon"
+ },
+ {
+ "name": "Adivarakhe",
+ "pos_x": 144.15625,
+ "pos_y": -35.96875,
+ "pos_z": -77,
+ "stations": "Hoshide Dock,Hinz Colony,Rotsler Depot"
+ },
+ {
+ "name": "Adivas",
+ "pos_x": -51.34375,
+ "pos_y": 80,
+ "pos_z": -67.46875,
+ "stations": "Hovell Terminal,Soto Terminal,Burgess Enterprise,Schilling Enterprise,Baffin Dock,Watts Station,Csoma Enterprise,Evans' Pride,Jones Legacy,White Works"
+ },
+ {
+ "name": "Adiyan",
+ "pos_x": 101.53125,
+ "pos_y": -131.53125,
+ "pos_z": 145.90625,
+ "stations": "Weill Camp"
+ },
+ {
+ "name": "Adju",
+ "pos_x": -14.375,
+ "pos_y": -24.25,
+ "pos_z": 144.25,
+ "stations": "Darnielle Gateway,Rowley Arena,Grego Landing,Moskowitz Keep"
+ },
+ {
+ "name": "Adjukru",
+ "pos_x": -20.09375,
+ "pos_y": 7.875,
+ "pos_z": -96.34375,
+ "stations": "von Helmont Base"
+ },
+ {
+ "name": "Adlirvantan",
+ "pos_x": 66.65625,
+ "pos_y": 88.90625,
+ "pos_z": 136.71875,
+ "stations": "Wagner Dock,Stasheff Vision"
+ },
+ {
+ "name": "Adlivun",
+ "pos_x": -176.9375,
+ "pos_y": -95.3125,
+ "pos_z": 21.5,
+ "stations": "Abe Survey"
+ },
+ {
+ "name": "Adlivuni",
+ "pos_x": 88.6875,
+ "pos_y": -21.1875,
+ "pos_z": 130.21875,
+ "stations": "Grushin Base,Bickel Settlement"
+ },
+ {
+ "name": "Adnoannoda",
+ "pos_x": -31.75,
+ "pos_y": -111.53125,
+ "pos_z": -92.6875,
+ "stations": "Bernoulli Gateway,Boyle Enterprise"
+ },
+ {
+ "name": "Adnoartina",
+ "pos_x": 101.84375,
+ "pos_y": -141.40625,
+ "pos_z": 48.125,
+ "stations": "Babcock Landing,Cleve Works,Kojima Hub"
+ },
+ {
+ "name": "Adnyan",
+ "pos_x": 140.34375,
+ "pos_y": 21.8125,
+ "pos_z": -15.84375,
+ "stations": "Drexler Platform,Blodgett Prospect"
+ },
+ {
+ "name": "Adnyayar",
+ "pos_x": 103.53125,
+ "pos_y": 65.0625,
+ "pos_z": -117.3125,
+ "stations": "Shiras Hub,Mitchison Port,Archer Horizons,Irens Port"
+ },
+ {
+ "name": "Adria",
+ "pos_x": 16.03125,
+ "pos_y": -64.96875,
+ "pos_z": -99.5,
+ "stations": "Goddard Horizons,Drexler Relay,Bertin Holdings,Drebbel Installation"
+ },
+ {
+ "name": "Adriatikuru",
+ "pos_x": -104.1875,
+ "pos_y": 35.53125,
+ "pos_z": 42.15625,
+ "stations": "Grimwood Gateway,Oliver Market,Menzies Gateway,Morrow Gateway,Griffiths Dock,Chadwick Horizons,Burton Penal colony"
+ },
+ {
+ "name": "Adro",
+ "pos_x": -130.4375,
+ "pos_y": -22.65625,
+ "pos_z": 11.96875,
+ "stations": "Cartier Refinery,Menezes Installation,Adams Enterprise"
+ },
+ {
+ "name": "ADS 10035",
+ "pos_x": 19.84375,
+ "pos_y": 24.40625,
+ "pos_z": 103.21875,
+ "stations": "Wisoff Ring,Burbank Dock,Vaugh Port"
+ },
+ {
+ "name": "ADS 16553",
+ "pos_x": -60.40625,
+ "pos_y": -53.96875,
+ "pos_z": -0.0625,
+ "stations": "Reiter Plant,Thornton Platform,Stevin Enterprise"
+ },
+ {
+ "name": "ADS 8444",
+ "pos_x": 70.125,
+ "pos_y": 74.40625,
+ "pos_z": 25.84375,
+ "stations": "Thoreau Station,Isherwood Holdings,Hieb Dock"
+ },
+ {
+ "name": "Aduni",
+ "pos_x": 122.1875,
+ "pos_y": 65.25,
+ "pos_z": 108.625,
+ "stations": "Young Port,Everest Orbital"
+ },
+ {
+ "name": "Aduninuan",
+ "pos_x": 43.8125,
+ "pos_y": -9.625,
+ "pos_z": -101.375,
+ "stations": "Nikolayev Prospect,Moore Arsenal,Stanier Hangar"
+ },
+ {
+ "name": "AE Fornacis",
+ "pos_x": 31.375,
+ "pos_y": -87.71875,
+ "pos_z": -42.96875,
+ "stations": "Deb Colony,Pelliot Vision,Avogadro Hub"
+ },
+ {
+ "name": "Aebocori",
+ "pos_x": 118,
+ "pos_y": -77.71875,
+ "pos_z": 80.0625,
+ "stations": "Barcelona Orbital,Barreiros Vision,Bickel Station"
+ },
+ {
+ "name": "Aebocorio",
+ "pos_x": 48.15625,
+ "pos_y": -228.25,
+ "pos_z": 72.5,
+ "stations": "Albitzky Port,Dolgov Camp,Ozanne Depot"
+ },
+ {
+ "name": "Aecerbot",
+ "pos_x": 66.375,
+ "pos_y": -10.78125,
+ "pos_z": -23.25,
+ "stations": "Lawson Survey,Bobko Dock"
+ },
+ {
+ "name": "Aeduci",
+ "pos_x": -138.40625,
+ "pos_y": 81.28125,
+ "pos_z": -12.09375,
+ "stations": "Manakov Survey,Julian Settlement"
+ },
+ {
+ "name": "Aeducini",
+ "pos_x": 197.78125,
+ "pos_y": -148.3125,
+ "pos_z": 37.8125,
+ "stations": "Herzog Prospect,Pittendreigh Prospect,Blish Camp,Doyle Beacon"
+ },
+ {
+ "name": "Aedui",
+ "pos_x": -113.90625,
+ "pos_y": -4.4375,
+ "pos_z": 103,
+ "stations": "Chu Base,Baudry Works"
+ },
+ {
+ "name": "Aeduwona",
+ "pos_x": 108.3125,
+ "pos_y": -165.84375,
+ "pos_z": 79.5,
+ "stations": "Nouvel Retreat,Brorsen Installation,Auwers Escape"
+ },
+ {
+ "name": "Aegaeon",
+ "pos_x": 46.90625,
+ "pos_y": 23.625,
+ "pos_z": -59.75,
+ "stations": "Aldrin Colony,Schweickart Station,Langford Plant,Savinykh Hub"
+ },
+ {
+ "name": "Aegida",
+ "pos_x": -14.09375,
+ "pos_y": -203.34375,
+ "pos_z": 43,
+ "stations": "Adams City,Payne-Scott City,Klink Vista,Titius Bastion,Thomson City"
+ },
+ {
+ "name": "Aegidal",
+ "pos_x": 127.65625,
+ "pos_y": 54.09375,
+ "pos_z": -81.65625,
+ "stations": "Chadwick Plant"
+ },
+ {
+ "name": "Aegilakan",
+ "pos_x": -3.53125,
+ "pos_y": 74.25,
+ "pos_z": -81.75,
+ "stations": "Potter Hub"
+ },
+ {
+ "name": "Aegilips",
+ "pos_x": 22.96875,
+ "pos_y": 28.9375,
+ "pos_z": 28.40625,
+ "stations": "Carr Silo,Bardeen Terminal"
+ },
+ {
+ "name": "Aegir",
+ "pos_x": -135.46875,
+ "pos_y": -8.6875,
+ "pos_z": 128.75,
+ "stations": "Grijalva Freeport"
+ },
+ {
+ "name": "Aeneas",
+ "pos_x": 54.40625,
+ "pos_y": 35.90625,
+ "pos_z": 24.9375,
+ "stations": "Russo Ring,Fanning Ring"
+ },
+ {
+ "name": "Aeng Dimo",
+ "pos_x": -45.78125,
+ "pos_y": -12.09375,
+ "pos_z": -21.59375,
+ "stations": "Puleston Terminal,Cernan Station,Boodt Hub,McArthur Port"
+ },
+ {
+ "name": "Aeolus",
+ "pos_x": -43.28125,
+ "pos_y": 37.28125,
+ "pos_z": -31.53125,
+ "stations": "Yu Station,Nelder Dock"
+ },
+ {
+ "name": "Aequeelg",
+ "pos_x": -80.125,
+ "pos_y": 25.4375,
+ "pos_z": 17.5,
+ "stations": "Hopkins Plant,Robinson Station"
+ },
+ {
+ "name": "Aequiatalaa",
+ "pos_x": 133.4375,
+ "pos_y": -191.46875,
+ "pos_z": 69,
+ "stations": "Isaev Terminal"
+ },
+ {
+ "name": "Aerial",
+ "pos_x": 100.75,
+ "pos_y": -102.625,
+ "pos_z": 8.40625,
+ "stations": "dhp11,Andrade Legacy,Lewitt Gateway,Watt-Evans Settlement"
+ },
+ {
+ "name": "Aeternitas",
+ "pos_x": -1.5,
+ "pos_y": -14.65625,
+ "pos_z": 56.875,
+ "stations": "Houtman City,Gentle Horizons,Filter Station,Binder Dock,Weyl Hub,Mitchell Arsenal,Lenthall Stop"
+ },
+ {
+ "name": "Aetius",
+ "pos_x": -64.6875,
+ "pos_y": -58.71875,
+ "pos_z": -26.03125,
+ "stations": "Forfait Platform"
+ },
+ {
+ "name": "AF Leporis",
+ "pos_x": 45.90625,
+ "pos_y": -35.1875,
+ "pos_z": -66.59375,
+ "stations": "Murray Platform,Vinci's Inheritance"
+ },
+ {
+ "name": "Afli",
+ "pos_x": 35.3125,
+ "pos_y": -78.03125,
+ "pos_z": 38.625,
+ "stations": "Pu City,Quetelet Dock,Bullialdus Palace,Skjellerup Vista,Reinhold Orbital,Thomas Ring,Zander Vision"
+ },
+ {
+ "name": "AG+11 1351",
+ "pos_x": 44.8125,
+ "pos_y": 108.84375,
+ "pos_z": -11.34375,
+ "stations": "Cochrane Terminal,Bradshaw Base"
+ },
+ {
+ "name": "AG+21 1754",
+ "pos_x": -53.8125,
+ "pos_y": 29.03125,
+ "pos_z": 50.71875,
+ "stations": "Aleksandrov's Claim,Oleskiw Hangar,Still Horizons"
+ },
+ {
+ "name": "Aganippe",
+ "pos_x": -11.5625,
+ "pos_y": 43.8125,
+ "pos_z": 11.625,
+ "stations": "Julian Market,Cherry Port,Vasilyev Port"
+ },
+ {
+ "name": "Agarahma",
+ "pos_x": -40.125,
+ "pos_y": 73.46875,
+ "pos_z": -154.15625,
+ "stations": "Hawke Terminal,Pike Works,Shaikh Mines,Barbosa Plant"
+ },
+ {
+ "name": "Agarda",
+ "pos_x": 75.21875,
+ "pos_y": 40.3125,
+ "pos_z": -30.09375,
+ "stations": "Stephenson Holdings,Rusch Enterprise,Loncke Vision,Kirtley Orbital"
+ },
+ {
+ "name": "Agarla",
+ "pos_x": 63.28125,
+ "pos_y": -115.375,
+ "pos_z": 83.5,
+ "stations": "Wilson Port,Weizsacker Hub,Moore Lab,Anderson City"
+ },
+ {
+ "name": "Agartha",
+ "pos_x": 70.71875,
+ "pos_y": -123.65625,
+ "pos_z": 41.3125,
+ "stations": "Cummings Resort,Enoch Port"
+ },
+ {
+ "name": "Agasa",
+ "pos_x": 26.25,
+ "pos_y": 51.71875,
+ "pos_z": -82.125,
+ "stations": "Gaspar de Portola Port,Baxter Laboratory"
+ },
+ {
+ "name": "Agaso",
+ "pos_x": -100.8125,
+ "pos_y": -58.21875,
+ "pos_z": 115.09375,
+ "stations": "Litke Landing"
+ },
+ {
+ "name": "Agasta",
+ "pos_x": 143.03125,
+ "pos_y": -105.5625,
+ "pos_z": 116.71875,
+ "stations": "Bracewell Holdings,McQuay Mines,Nilson Prospect"
+ },
+ {
+ "name": "Agastani",
+ "pos_x": 22.375,
+ "pos_y": -17.6875,
+ "pos_z": -7.78125,
+ "stations": "Vesalius Terminal,Leslie City,Bell Terminal,Mitchell Enterprise,Battuta Dock,Oppenheimer Keep"
+ },
+ {
+ "name": "Agastariya",
+ "pos_x": 34.625,
+ "pos_y": -104.84375,
+ "pos_z": 16.15625,
+ "stations": "Biruni Gateway,al-Kashi's Inheritance,Kingsmill Penal colony"
+ },
+ {
+ "name": "Agastii",
+ "pos_x": -57.0625,
+ "pos_y": -120.53125,
+ "pos_z": -68.59375,
+ "stations": "Beebe's Claim"
+ },
+ {
+ "name": "Agastja",
+ "pos_x": -73.84375,
+ "pos_y": 128.53125,
+ "pos_z": -7.96875,
+ "stations": "Ziemianski Ring,Leiber Port,Alpers Installation"
+ },
+ {
+ "name": "Agat Yu",
+ "pos_x": 101.96875,
+ "pos_y": 19.09375,
+ "pos_z": -17.75,
+ "stations": "Cortes Beacon"
+ },
+ {
+ "name": "Agatal",
+ "pos_x": 83.84375,
+ "pos_y": 122.65625,
+ "pos_z": 14.46875,
+ "stations": "Guidoni Vision"
+ },
+ {
+ "name": "Agatavun",
+ "pos_x": 58.375,
+ "pos_y": 33.125,
+ "pos_z": 62.15625,
+ "stations": "Tuan Station,Grandin Plant,Melvill Holdings,Reamy Refinery"
+ },
+ {
+ "name": "Agathiar",
+ "pos_x": -17.84375,
+ "pos_y": 59.40625,
+ "pos_z": 66.875,
+ "stations": "Haipeng Dock,Potrykus Orbital,Linnaeus Enterprise"
+ },
+ {
+ "name": "Ageno",
+ "pos_x": -50.9375,
+ "pos_y": -48.09375,
+ "pos_z": -99.875,
+ "stations": "Pook Dock,Marlowe Base"
+ },
+ {
+ "name": "AGKR 1017",
+ "pos_x": -53.5,
+ "pos_y": -85.375,
+ "pos_z": -47.9375,
+ "stations": "Kimberlin Refinery,Powell Mine,Citi Settlement"
+ },
+ {
+ "name": "Aguan",
+ "pos_x": 47.59375,
+ "pos_y": 66.6875,
+ "pos_z": 116.96875,
+ "stations": "Person Base,Davidson Colony,Shunn Platform"
+ },
+ {
+ "name": "Aguana",
+ "pos_x": 82.875,
+ "pos_y": 135.09375,
+ "pos_z": 81.90625,
+ "stations": "Webb Dock,Desargues Hub"
+ },
+ {
+ "name": "Aguane",
+ "pos_x": -63.84375,
+ "pos_y": 67.21875,
+ "pos_z": 88.28125,
+ "stations": "Williams Colony"
+ },
+ {
+ "name": "Aguano",
+ "pos_x": 73.40625,
+ "pos_y": -103,
+ "pos_z": 115.28125,
+ "stations": "Dyr Beacon,Bartolomeu de Gusmao Orbital,Borrelly Dock,Dahm Port,Dufay Dock,Shinjo Terminal,Salam Bastion,Cochrane Installation"
+ },
+ {
+ "name": "Aguaroing",
+ "pos_x": 57.53125,
+ "pos_y": -39.1875,
+ "pos_z": 141.625,
+ "stations": "Stebbins Colony"
+ },
+ {
+ "name": "Agulmanji",
+ "pos_x": 153.375,
+ "pos_y": -63.1875,
+ "pos_z": 44.09375,
+ "stations": "Swift Base,Penrose's Pride"
+ },
+ {
+ "name": "Agulmasci",
+ "pos_x": -17.84375,
+ "pos_y": -30.375,
+ "pos_z": 118.0625,
+ "stations": "Wallis Arena"
+ },
+ {
+ "name": "Agulu",
+ "pos_x": 93.3125,
+ "pos_y": -242.46875,
+ "pos_z": 60,
+ "stations": "Bauschinger Dock,Prandtl's Claim"
+ },
+ {
+ "name": "Agwa",
+ "pos_x": 6.9375,
+ "pos_y": 20.3125,
+ "pos_z": -156.90625,
+ "stations": "Bryant Enterprise,Godel Station,Ing Settlement"
+ },
+ {
+ "name": "Agwe",
+ "pos_x": -48.5625,
+ "pos_y": 47.53125,
+ "pos_z": -49.125,
+ "stations": "Sadi Carnot City,Hutton Dock,Hedley Penal colony,Cassidy Platform,Al-Battani Relay"
+ },
+ {
+ "name": "Aha Narfi",
+ "pos_x": -80.5,
+ "pos_y": -58.65625,
+ "pos_z": 96.125,
+ "stations": "Lem Station"
+ },
+ {
+ "name": "Aha Wa",
+ "pos_x": 90.375,
+ "pos_y": -56.625,
+ "pos_z": -17.5625,
+ "stations": "Searle Survey,Marth Station"
+ },
+ {
+ "name": "Ahan",
+ "pos_x": -60.3125,
+ "pos_y": 79,
+ "pos_z": 140.625,
+ "stations": "Schlegel Settlement,Sanger Settlement,Vetulani Base"
+ },
+ {
+ "name": "Ahanla",
+ "pos_x": 60.90625,
+ "pos_y": -215.25,
+ "pos_z": 83.03125,
+ "stations": "Lagerkvist Prospect,al-Din al-Urdi Landing"
+ },
+ {
+ "name": "Ahanyamwezi",
+ "pos_x": -95.78125,
+ "pos_y": -42.34375,
+ "pos_z": 99.5,
+ "stations": "Pailes Relay,Gauss Prospect"
+ },
+ {
+ "name": "Ahau Kichua",
+ "pos_x": -53.25,
+ "pos_y": 130.96875,
+ "pos_z": 23.71875,
+ "stations": "Asprin Arena"
+ },
+ {
+ "name": "Ahaudheim",
+ "pos_x": -29.25,
+ "pos_y": 123.96875,
+ "pos_z": -124.0625,
+ "stations": "Zelazny Prospect,Normand Colony"
+ },
+ {
+ "name": "Ahaudhr",
+ "pos_x": 137.9375,
+ "pos_y": -71.03125,
+ "pos_z": -48.65625,
+ "stations": "Lomonosov Horizons,Silverberg Silo"
+ },
+ {
+ "name": "Ahauduwonai",
+ "pos_x": 93.1875,
+ "pos_y": -84.53125,
+ "pos_z": -74.96875,
+ "stations": "Ali Terminal,Cenker Terminal,Krikalev Station,Coppel Landing"
+ },
+ {
+ "name": "Ahaurawai",
+ "pos_x": -106.96875,
+ "pos_y": -20.15625,
+ "pos_z": 40.5625,
+ "stations": "Mattingly Dock,Cooper Settlement"
+ },
+ {
+ "name": "Ahaut",
+ "pos_x": 51.21875,
+ "pos_y": -246.125,
+ "pos_z": -42.15625,
+ "stations": "Goldberg Orbital,Kludze Terminal,Forbes Vision"
+ },
+ {
+ "name": "Ahauts",
+ "pos_x": -90.65625,
+ "pos_y": -142.9375,
+ "pos_z": 83.3125,
+ "stations": "Clark Dock,Fadlan Terminal,Vasilyev Enterprise"
+ },
+ {
+ "name": "Ahayan",
+ "pos_x": 6.3125,
+ "pos_y": -44.4375,
+ "pos_z": 157.84375,
+ "stations": "Goeppert-Mayer Vision,Feustel Ring,Pascal Platform"
+ },
+ {
+ "name": "Ahaykenkan",
+ "pos_x": -70.3125,
+ "pos_y": -176.28125,
+ "pos_z": -78.84375,
+ "stations": "Kirk Base,Boucher Dock"
+ },
+ {
+ "name": "Ahayus",
+ "pos_x": -36.625,
+ "pos_y": 107.59375,
+ "pos_z": 109.5,
+ "stations": "Alvarado Beacon,Vercors Outpost,Tilman Works"
+ },
+ {
+ "name": "Ahemaker",
+ "pos_x": 18.8125,
+ "pos_y": -70.03125,
+ "pos_z": 143.71875,
+ "stations": "De Lay Survey"
+ },
+ {
+ "name": "Ahemakino",
+ "pos_x": 123.25,
+ "pos_y": -3.21875,
+ "pos_z": -97.4375,
+ "stations": "Mattingly Port,Pailes Camp,Haarsma Keep"
+ },
+ {
+ "name": "Ahemez",
+ "pos_x": -103.34375,
+ "pos_y": -84.6875,
+ "pos_z": -75.875,
+ "stations": "Rennie Survey,Griggs Terminal,Baudry Hub,Hedin Enterprise,Blaylock Enterprise"
+ },
+ {
+ "name": "Ahmetes",
+ "pos_x": 116.5625,
+ "pos_y": -96.71875,
+ "pos_z": 89.625,
+ "stations": "Beg Terminal"
+ },
+ {
+ "name": "Ahmeti",
+ "pos_x": 167.09375,
+ "pos_y": -104.78125,
+ "pos_z": 5.21875,
+ "stations": "Jean Colony,Niijima Landing,Ellern Base"
+ },
+ {
+ "name": "Ahol",
+ "pos_x": 164.375,
+ "pos_y": -76.125,
+ "pos_z": -34.125,
+ "stations": "Vess Landing,Antonio de Andrade's Claim,Hatanaka Colony"
+ },
+ {
+ "name": "Aholetondal",
+ "pos_x": 1.9375,
+ "pos_y": -123.5625,
+ "pos_z": 93,
+ "stations": "Borrego Gateway"
+ },
+ {
+ "name": "Aholhyniai",
+ "pos_x": 53.90625,
+ "pos_y": -128.3125,
+ "pos_z": -56.5625,
+ "stations": "Bessel Terminal,Stuart Keep,Roche Dock,Kaneda Dock,Erikson Town"
+ },
+ {
+ "name": "Ahpuchan",
+ "pos_x": 40.71875,
+ "pos_y": -159.25,
+ "pos_z": 147,
+ "stations": "Barnwell Platform,Zetford Observatory"
+ },
+ {
+ "name": "Ahti",
+ "pos_x": 4.0625,
+ "pos_y": -79.09375,
+ "pos_z": -7.71875,
+ "stations": "Rushd Holdings"
+ },
+ {
+ "name": "Aiaba",
+ "pos_x": 97.3125,
+ "pos_y": 51.625,
+ "pos_z": 103.125,
+ "stations": "Vespucci City,Denton Installation"
+ },
+ {
+ "name": "Aiaban",
+ "pos_x": -21.5625,
+ "pos_y": -201.34375,
+ "pos_z": 79,
+ "stations": "Wright Mines,Heinkel Mines"
+ },
+ {
+ "name": "Aiabara",
+ "pos_x": -13.09375,
+ "pos_y": -32.125,
+ "pos_z": 85.4375,
+ "stations": "Grechko Prospect"
+ },
+ {
+ "name": "Aiabiko",
+ "pos_x": -82.0625,
+ "pos_y": 1.625,
+ "pos_z": -14.4375,
+ "stations": "Gooch Terminal,White Orbital,Jett Ring,Wollheim Holdings,Treshchov Port,Maxwell Orbital,Haisheng Port,Savitskaya Station,Haber Dock,Scheerbart Beacon,Weber Installation,Weston Dock,Williams Hub"
+ },
+ {
+ "name": "Aiga",
+ "pos_x": 66.625,
+ "pos_y": -147.5625,
+ "pos_z": 102.78125,
+ "stations": "Dahm Port,Mitchison's Claim"
+ },
+ {
+ "name": "Aigaha",
+ "pos_x": 73.34375,
+ "pos_y": -99.75,
+ "pos_z": -97.25,
+ "stations": "Qushji Base"
+ },
+ {
+ "name": "Aigalie",
+ "pos_x": 166.4375,
+ "pos_y": -76.25,
+ "pos_z": -1.6875,
+ "stations": "Truly Prospect,Adams Enterprise,Wolf Point"
+ },
+ {
+ "name": "Aigones",
+ "pos_x": -123.15625,
+ "pos_y": 29.59375,
+ "pos_z": 101.5,
+ "stations": "Card Vision"
+ },
+ {
+ "name": "Aika",
+ "pos_x": 12.46875,
+ "pos_y": -210.9375,
+ "pos_z": 94.53125,
+ "stations": "Penrose's Claim,De Lay Beacon"
+ },
+ {
+ "name": "Ailure",
+ "pos_x": -92.625,
+ "pos_y": 30.75,
+ "pos_z": 157.46875,
+ "stations": "Cochrane Stop,Weyl Terminal,Katzenstein Base"
+ },
+ {
+ "name": "Ailuremowo",
+ "pos_x": 89.0625,
+ "pos_y": 14.8125,
+ "pos_z": -141.15625,
+ "stations": "Piserchia Port,Kotzebue Survey,Kettle Station,Gardner Forum,Turtledove Settlement"
+ },
+ {
+ "name": "Ailurians",
+ "pos_x": 96.71875,
+ "pos_y": 24.90625,
+ "pos_z": 47.71875,
+ "stations": "Reiter Vision,Broglie Port,Zhigang Orbital"
+ },
+ {
+ "name": "Ailurii",
+ "pos_x": 56.375,
+ "pos_y": -125.28125,
+ "pos_z": 157.0625,
+ "stations": "Hussenot Vision"
+ },
+ {
+ "name": "Ailurui",
+ "pos_x": 95.34375,
+ "pos_y": -3.5,
+ "pos_z": 23.5625,
+ "stations": "Piercy Hub,Yu Barracks,Younghusband Terminal"
+ },
+ {
+ "name": "Aimo Jung",
+ "pos_x": -9.90625,
+ "pos_y": 81.59375,
+ "pos_z": -63.8125,
+ "stations": "Lloyd Settlement,Barr's Inheritance"
+ },
+ {
+ "name": "Ainuan",
+ "pos_x": -142.5625,
+ "pos_y": 63.5,
+ "pos_z": 5.59375,
+ "stations": "de Caminha Dock,Shirley Dock,Bayley Terminal,King Vision"
+ },
+ {
+ "name": "Ainun",
+ "pos_x": 125.84375,
+ "pos_y": 49.375,
+ "pos_z": 2.03125,
+ "stations": "Fife Settlement,George Settlement,Herjulfsson's Pride"
+ },
+ {
+ "name": "Ainunnicori",
+ "pos_x": -24.5,
+ "pos_y": -52.625,
+ "pos_z": -77.03125,
+ "stations": "Tani Hub,Sinclair Port,Ansari Enterprise,Stefansson's Pride"
+ },
+ {
+ "name": "Ainuro",
+ "pos_x": -62.3125,
+ "pos_y": 28.375,
+ "pos_z": 117.5625,
+ "stations": "Shirley Reach,Toll Landing"
+ },
+ {
+ "name": "Airman Di",
+ "pos_x": -72.8125,
+ "pos_y": -15.65625,
+ "pos_z": -115.65625,
+ "stations": "Chargaff Orbital,Timofeyevich Settlement,Fourneyron Port,Metcalf Hub"
+ },
+ {
+ "name": "Airmang",
+ "pos_x": -22.8125,
+ "pos_y": 107.6875,
+ "pos_z": 132.40625,
+ "stations": "Burbank Depot"
+ },
+ {
+ "name": "Airs",
+ "pos_x": -115.28125,
+ "pos_y": 7.53125,
+ "pos_z": 71.3125,
+ "stations": "Asaro Landing,Pelt City,Gulyaev Depot"
+ },
+ {
+ "name": "Airseklis",
+ "pos_x": 194.34375,
+ "pos_y": -116.15625,
+ "pos_z": 7.3125,
+ "stations": "Fokker Prospect,Levi-Civita Depot"
+ },
+ {
+ "name": "Airsetanoa",
+ "pos_x": 103.09375,
+ "pos_y": -38.8125,
+ "pos_z": 32.1875,
+ "stations": "Leavitt Hub,Halley Hub,Baturin Orbital"
+ },
+ {
+ "name": "Aisoci",
+ "pos_x": 124.1875,
+ "pos_y": -162.875,
+ "pos_z": 103.59375,
+ "stations": "Emshwiller Port,Molchanov Point,Gromov Base,Lagrange Base"
+ },
+ {
+ "name": "Aisociti",
+ "pos_x": 82.71875,
+ "pos_y": 38.53125,
+ "pos_z": 111.65625,
+ "stations": "Hugh Reformatory"
+ },
+ {
+ "name": "Aisoyiman",
+ "pos_x": -31.71875,
+ "pos_y": 65.09375,
+ "pos_z": 93.15625,
+ "stations": "Satcher Works,Schirra Dock"
+ },
+ {
+ "name": "Aitva",
+ "pos_x": 19.0625,
+ "pos_y": -214.25,
+ "pos_z": 11,
+ "stations": "Kandrup Vision"
+ },
+ {
+ "name": "Aitvanec",
+ "pos_x": 42.15625,
+ "pos_y": 115.3125,
+ "pos_z": 10.9375,
+ "stations": "Julian Gateway,Shosuke Gateway,Chorel Landing,Reed Horizons"
+ },
+ {
+ "name": "Aitvani",
+ "pos_x": 161.25,
+ "pos_y": -56.25,
+ "pos_z": -33.21875,
+ "stations": "Hartmann Bastion,Potocnik Platform,Wood Settlement"
+ },
+ {
+ "name": "Aitvaniez",
+ "pos_x": 100.09375,
+ "pos_y": -100,
+ "pos_z": -62.375,
+ "stations": "Schiaparelli Station"
+ },
+ {
+ "name": "Aitvara",
+ "pos_x": -31.0625,
+ "pos_y": -18.53125,
+ "pos_z": 112.28125,
+ "stations": "Manarov Platform,Macarthur Dock"
+ },
+ {
+ "name": "Aitvaredd",
+ "pos_x": -55.46875,
+ "pos_y": -102.71875,
+ "pos_z": -62.78125,
+ "stations": "Chalker Station,Meyrink Ring,Lagrange Horizons,Davis Settlement"
+ },
+ {
+ "name": "Aitvas",
+ "pos_x": 18.46875,
+ "pos_y": -202.21875,
+ "pos_z": -62.09375,
+ "stations": "Flettner Survey,Tomita Enterprise"
+ },
+ {
+ "name": "Ajabog",
+ "pos_x": 157.375,
+ "pos_y": 43.96875,
+ "pos_z": -1.125,
+ "stations": "Schlegel Station"
+ },
+ {
+ "name": "Ajabon",
+ "pos_x": -28.4375,
+ "pos_y": 6.46875,
+ "pos_z": 159.90625,
+ "stations": "Garrett Hub,Bertin Station,Narita Gateway,Matthews Relay"
+ },
+ {
+ "name": "Ajani",
+ "pos_x": 129.4375,
+ "pos_y": -98.125,
+ "pos_z": 15.625,
+ "stations": "Narlikar Survey"
+ },
+ {
+ "name": "Aje",
+ "pos_x": -178.6875,
+ "pos_y": -9.1875,
+ "pos_z": -32.0625,
+ "stations": "Savery Dock,Kopra Dock"
+ },
+ {
+ "name": "Aje Shaluga",
+ "pos_x": 173.21875,
+ "pos_y": -72.03125,
+ "pos_z": 10.5625,
+ "stations": "al-Kashi Port"
+ },
+ {
+ "name": "Aje Shen",
+ "pos_x": -104.84375,
+ "pos_y": 12.78125,
+ "pos_z": 136.34375,
+ "stations": "Lehtonen Depot,Schouten Holdings"
+ },
+ {
+ "name": "Ajoku",
+ "pos_x": 56.46875,
+ "pos_y": -250.0625,
+ "pos_z": -63.84375,
+ "stations": "Porges Camp,Pearse Landing,Havilland Horizons,Otus Installation"
+ },
+ {
+ "name": "Aka",
+ "pos_x": -1.6875,
+ "pos_y": 168.75,
+ "pos_z": 57.46875,
+ "stations": "Wilson Landing"
+ },
+ {
+ "name": "Akanago",
+ "pos_x": 3.28125,
+ "pos_y": -36.125,
+ "pos_z": 86.9375,
+ "stations": "Thomson Station,Boole Escape,Nicollet Oasis"
+ },
+ {
+ "name": "Akandi",
+ "pos_x": 62.03125,
+ "pos_y": 10.75,
+ "pos_z": 38.875,
+ "stations": "Gessi Vista,Agnesi Colony"
+ },
+ {
+ "name": "Akandinigua",
+ "pos_x": 85.65625,
+ "pos_y": -18.15625,
+ "pos_z": -4.5625,
+ "stations": "Diophantus Prospect,Harrison Holdings,Rusch Station,Tiliala's Lament"
+ },
+ {
+ "name": "Akarn",
+ "pos_x": 28.46875,
+ "pos_y": 37.625,
+ "pos_z": 80.28125,
+ "stations": "Newton Station,Lobachevsky Beacon"
+ },
+ {
+ "name": "Akba Achih",
+ "pos_x": 119,
+ "pos_y": -98.28125,
+ "pos_z": -56.40625,
+ "stations": "Condit Survey"
+ },
+ {
+ "name": "Akba Atacab",
+ "pos_x": -119.46875,
+ "pos_y": 42.75,
+ "pos_z": 80.34375,
+ "stations": "Jones City,Legendre Prospect,Johnson Plant,Jun Base,Santarem Holdings"
+ },
+ {
+ "name": "Akba Ataci",
+ "pos_x": -36.125,
+ "pos_y": -73.40625,
+ "pos_z": 49.21875,
+ "stations": "Conrad Platform"
+ },
+ {
+ "name": "Akbakara",
+ "pos_x": -88.28125,
+ "pos_y": 53.71875,
+ "pos_z": -71.5625,
+ "stations": "Bennett Horizons,Herreshoff Base,Fairbairn Platform,Cook Landing"
+ },
+ {
+ "name": "Akbaksas",
+ "pos_x": -115.0625,
+ "pos_y": 78.59375,
+ "pos_z": 40.59375,
+ "stations": "Reynolds Mines"
+ },
+ {
+ "name": "Akbakshu",
+ "pos_x": 141.96875,
+ "pos_y": -193.625,
+ "pos_z": 120.03125,
+ "stations": "Kosai Relay"
+ },
+ {
+ "name": "Akbulun",
+ "pos_x": -47,
+ "pos_y": 1.09375,
+ "pos_z": 80.15625,
+ "stations": "Sevastyanov Beacon,Levchenko Dock,Birdseye Colony"
+ },
+ {
+ "name": "Aken",
+ "pos_x": 17.71875,
+ "pos_y": 98.90625,
+ "pos_z": 119.40625,
+ "stations": "Hedin Prospect,Vasquez de Coronado Colony,Schmitz Enterprise"
+ },
+ {
+ "name": "Akena Buj",
+ "pos_x": -129.90625,
+ "pos_y": 68,
+ "pos_z": 55.625,
+ "stations": "Langford Reach,Cavalieri Survey"
+ },
+ {
+ "name": "Akenata",
+ "pos_x": -18.78125,
+ "pos_y": 115.6875,
+ "pos_z": 85.0625,
+ "stations": "Hoshide Depot,Feynman Platform"
+ },
+ {
+ "name": "Akenates",
+ "pos_x": 155.25,
+ "pos_y": -182.15625,
+ "pos_z": 39.25,
+ "stations": "Wegner Port,Asher Bastion,Furuta Vision"
+ },
+ {
+ "name": "Aker",
+ "pos_x": 89.5,
+ "pos_y": -103.0625,
+ "pos_z": 116.625,
+ "stations": "Milne Vision,Veron Park,Jeans Penal colony,Barnes Base,Lassell Installation"
+ },
+ {
+ "name": "Akeretia",
+ "pos_x": -17.5625,
+ "pos_y": 84.625,
+ "pos_z": 105.03125,
+ "stations": "Ozanne Estate,Alpers Orbital"
+ },
+ {
+ "name": "Akha Kacha",
+ "pos_x": 77.3125,
+ "pos_y": 70.5,
+ "pos_z": 77.0625,
+ "stations": "Frobisher Mine"
+ },
+ {
+ "name": "Akhabakti",
+ "pos_x": 24.3125,
+ "pos_y": -157.1875,
+ "pos_z": -51.6875,
+ "stations": "Grushin Vision,Bethke Horizons"
+ },
+ {
+ "name": "Akheilos",
+ "pos_x": -36.15625,
+ "pos_y": 69.625,
+ "pos_z": 13.21875,
+ "stations": "Smith City,Pawelczyk Orbital,Kettle Holdings,Titov Colony"
+ },
+ {
+ "name": "Akhenaten",
+ "pos_x": -53.375,
+ "pos_y": 7.84375,
+ "pos_z": -40.375,
+ "stations": "JEPOCHAL-G OUTPOST,Lee Station"
+ },
+ {
+ "name": "Akhvaroua",
+ "pos_x": 164.4375,
+ "pos_y": -116.28125,
+ "pos_z": -19.59375,
+ "stations": "Bohrmann Terminal"
+ },
+ {
+ "name": "Akhvathaang",
+ "pos_x": 37.46875,
+ "pos_y": 81.6875,
+ "pos_z": -43.21875,
+ "stations": "Lister Gateway"
+ },
+ {
+ "name": "Akkadia",
+ "pos_x": -1.75,
+ "pos_y": -33.90625,
+ "pos_z": -32.96875,
+ "stations": "Valdes Colony,Hotel Vogelsang Outpost,Calvin Lawrence,Buffett Vista,Kondakova Mines"
+ },
+ {
+ "name": "Aknandan",
+ "pos_x": -1.1875,
+ "pos_y": 76.3125,
+ "pos_z": 122.34375,
+ "stations": "Aksyonov Terminal,Pailes Orbital,Parry's Inheritance,Hurley Station,Wundt Terminal,Kondratyev Station,Gordon Terminal,Stanier Keep"
+ },
+ {
+ "name": "Aknango",
+ "pos_x": -155.40625,
+ "pos_y": 30,
+ "pos_z": -76.4375,
+ "stations": "Pinto Hub,Skolem Landing,Kippax Landing,Moorcock Settlement,Bischoff Dock"
+ },
+ {
+ "name": "Aknara",
+ "pos_x": 147.6875,
+ "pos_y": 14.90625,
+ "pos_z": -33.5,
+ "stations": "Witt Landing"
+ },
+ {
+ "name": "Aknata",
+ "pos_x": -88.8125,
+ "pos_y": -26.40625,
+ "pos_z": 108.84375,
+ "stations": "Thomas Stop"
+ },
+ {
+ "name": "Akondaluk",
+ "pos_x": 161.0625,
+ "pos_y": -57.8125,
+ "pos_z": 25.84375,
+ "stations": "Stiles Platform,Kibalchich Refinery,Lemaitre Observatory"
+ },
+ {
+ "name": "Akondos",
+ "pos_x": 98.21875,
+ "pos_y": -181.1875,
+ "pos_z": -21.625,
+ "stations": "Brothers Platform,Johnson's Folly"
+ },
+ {
+ "name": "Akonito",
+ "pos_x": -86.625,
+ "pos_y": 1.21875,
+ "pos_z": -79.46875,
+ "stations": "Tanner Enterprise,Vlamingh Installation,Chargaff Market,Bell Terminal,Cramer Hub"
+ },
+ {
+ "name": "Akonome",
+ "pos_x": 29.9375,
+ "pos_y": 117.96875,
+ "pos_z": 95,
+ "stations": "Cogswell Port,Al-Kashi Enterprise,Shatner Terminal,MacLeod's Folly"
+ },
+ {
+ "name": "Aktzin",
+ "pos_x": -51.78125,
+ "pos_y": 23.9375,
+ "pos_z": -29.40625,
+ "stations": "Hermite Vision,Feoktistov Station,Knight Dock,Wallace Orbital,Hirase Base"
+ },
+ {
+ "name": "Aku",
+ "pos_x": 53.8125,
+ "pos_y": 29.5,
+ "pos_z": 67.40625,
+ "stations": "Kaleri Terminal,Al Saud Point"
+ },
+ {
+ "name": "Akua",
+ "pos_x": 18.9375,
+ "pos_y": 80.375,
+ "pos_z": 118.40625,
+ "stations": "McMahon Horizons,Selous Dock,Lee's Progress,Nolan Base"
+ },
+ {
+ "name": "Akualanu",
+ "pos_x": 63.78125,
+ "pos_y": -128.5,
+ "pos_z": 3,
+ "stations": "Hughes Vista"
+ },
+ {
+ "name": "Akuapem",
+ "pos_x": 8.28125,
+ "pos_y": -157.5,
+ "pos_z": -76.46875,
+ "stations": "Lundmark Station,Harvia Station,Ponce de Leon Bastion"
+ },
+ {
+ "name": "Akuariks",
+ "pos_x": 126.53125,
+ "pos_y": -63.5,
+ "pos_z": -62.1875,
+ "stations": "Vernadsky Station,Pirsan Hub,Jensen Settlement,Papin Landing,Akers Gateway"
+ },
+ {
+ "name": "Akuni",
+ "pos_x": -78.34375,
+ "pos_y": 20.40625,
+ "pos_z": -66.25,
+ "stations": "Vinci Orbital,Conway Station,Dzhanibekov Hub,Miletus Arsenal,Disch Landing"
+ },
+ {
+ "name": "Akunta",
+ "pos_x": 117.65625,
+ "pos_y": -202.0625,
+ "pos_z": -0.78125,
+ "stations": "Messerschmitt Orbital,Skiff Platform,Toll Terminal"
+ },
+ {
+ "name": "Akuntsu",
+ "pos_x": 155.3125,
+ "pos_y": 2.9375,
+ "pos_z": 4.875,
+ "stations": "Wylie Dock,Tennyson d'Eyncourt Landing,Julian Freeport,Williams Enterprise,Glazkov Depot"
+ },
+ {
+ "name": "Al Mina",
+ "pos_x": 14.4375,
+ "pos_y": 43.78125,
+ "pos_z": -13.25,
+ "stations": "Nagel Hub,Manakov City,Tyson Hub,Gillekens Terminal,Furukawa Orbital,Chu Dock,Barratt Orbital,Karl Diesel Relay"
+ },
+ {
+ "name": "Al-Qaum",
+ "pos_x": -8.03125,
+ "pos_y": -13.96875,
+ "pos_z": -69.6875,
+ "stations": "Duke Hub,Shepard Ring,Cormack Orbital,Curie Depot"
+ },
+ {
+ "name": "Alacagui",
+ "pos_x": 63.65625,
+ "pos_y": -119,
+ "pos_z": 17.0625,
+ "stations": "Giancola Bastion,Husband Refinery,Nahavandi Platform"
+ },
+ {
+ "name": "Alacarakmo",
+ "pos_x": -32.40625,
+ "pos_y": 169.53125,
+ "pos_z": -49.4375,
+ "stations": "Weyl Gateway,Vlamingh Port,Julian Survey,Farkas Depot"
+ },
+ {
+ "name": "Alacha",
+ "pos_x": 80.25,
+ "pos_y": -220.71875,
+ "pos_z": 55.625,
+ "stations": "Laing Installation,Langley Settlement,Naburimannu Station"
+ },
+ {
+ "name": "Alads",
+ "pos_x": -136.71875,
+ "pos_y": -68.15625,
+ "pos_z": -34.1875,
+ "stations": "Pavlou Beacon,Wingrove's Folly"
+ },
+ {
+ "name": "Aladshes",
+ "pos_x": -121.21875,
+ "pos_y": -24.84375,
+ "pos_z": -117.96875,
+ "stations": "Rusch Platform,Menzies Dock,Shinn Barracks"
+ },
+ {
+ "name": "Alag",
+ "pos_x": -141.8125,
+ "pos_y": 95.03125,
+ "pos_z": 13.96875,
+ "stations": "Elcano Depot"
+ },
+ {
+ "name": "Alanaribri",
+ "pos_x": -32.84375,
+ "pos_y": -75.5625,
+ "pos_z": -94.0625,
+ "stations": "Musa al-Khwarizmi Works"
+ },
+ {
+ "name": "Alano",
+ "pos_x": 84.21875,
+ "pos_y": -49.5625,
+ "pos_z": 30.03125,
+ "stations": "Leestma Port,Bondar City,Walker Enterprise,Walters Legacy,Forrester Colony"
+ },
+ {
+ "name": "Alanto",
+ "pos_x": -128,
+ "pos_y": 44.90625,
+ "pos_z": 144.40625,
+ "stations": "Aguirre Mines"
+ },
+ {
+ "name": "Alaw",
+ "pos_x": -30.6875,
+ "pos_y": 69.96875,
+ "pos_z": 100.15625,
+ "stations": "Garden Dock"
+ },
+ {
+ "name": "Alawaiteri",
+ "pos_x": 139.09375,
+ "pos_y": 34.53125,
+ "pos_z": 24.125,
+ "stations": "MacCurdy Landing"
+ },
+ {
+ "name": "Alawannaq",
+ "pos_x": -125.53125,
+ "pos_y": 36.46875,
+ "pos_z": 21.84375,
+ "stations": "Broderick Port,Egan Silo,Moorcock Prospect,Crown Installation"
+ },
+ {
+ "name": "Alawongli",
+ "pos_x": -34.4375,
+ "pos_y": -57.25,
+ "pos_z": 47.5,
+ "stations": "Bunsen Plant,Remek Port"
+ },
+ {
+ "name": "Albangage",
+ "pos_x": 82.375,
+ "pos_y": 25.9375,
+ "pos_z": 25.8125,
+ "stations": "Bennett Port,Covey Enterprise,Bernoulli Station,Normand Holdings"
+ },
+ {
+ "name": "Albangait",
+ "pos_x": 96.78125,
+ "pos_y": -63.625,
+ "pos_z": 20.71875,
+ "stations": "Lee Orbital,Qureshi Terminal,van Houten Gateway"
+ },
+ {
+ "name": "Albango",
+ "pos_x": 24.09375,
+ "pos_y": -76.75,
+ "pos_z": 105.59375,
+ "stations": "Eschbach Landing,Tan Orbital,Scortia Point"
+ },
+ {
+ "name": "Albardhas",
+ "pos_x": -39.875,
+ "pos_y": -103.46875,
+ "pos_z": 112.125,
+ "stations": "Taylor Platform,Pike Settlement,Burstein Installation"
+ },
+ {
+ "name": "Albarib",
+ "pos_x": 96.4375,
+ "pos_y": -0.65625,
+ "pos_z": 25.5625,
+ "stations": "Lorentz Orbital,Artsebarsky Exchange,Robson Settlement,Hale Orbital,Tolstoi Beacon,Newcomen Port,Hadfield Station,Edison Hub,Mayr Station,Northrop Terminal,Aubakirov Hub,Wait Colburn Depot,Cheli Terminal,Gessi Terminal,Allen's Folly"
+ },
+ {
+ "name": "Albassapic",
+ "pos_x": 130.15625,
+ "pos_y": -65.1875,
+ "pos_z": 83.5,
+ "stations": "Trujillo Colony"
+ },
+ {
+ "name": "Albast",
+ "pos_x": -40.5625,
+ "pos_y": -65.96875,
+ "pos_z": -21.03125,
+ "stations": "Tanner Vision,Kuipers Horizons,Gagarin City,Cauchy Barracks,Taylor Horizons"
+ },
+ {
+ "name": "Alberta",
+ "pos_x": -9536.96875,
+ "pos_y": -917.25,
+ "pos_z": 19793.03125,
+ "stations": "Kraft Works,Berman Market"
+ },
+ {
+ "name": "Albicevci",
+ "pos_x": 65.875,
+ "pos_y": -11.5,
+ "pos_z": 42.90625,
+ "stations": "Dobrovolski Survey,Fraas Depot,Lysenko Mine"
+ },
+ {
+ "name": "Albienses",
+ "pos_x": 125.5,
+ "pos_y": -107.625,
+ "pos_z": 88.21875,
+ "stations": "Pons Station"
+ },
+ {
+ "name": "Albieteroo",
+ "pos_x": 107.5,
+ "pos_y": 68.46875,
+ "pos_z": 45.875,
+ "stations": "Newman Plant,McDaniel's Exile,Herzfeld Port"
+ },
+ {
+ "name": "Albisaki",
+ "pos_x": 68.21875,
+ "pos_y": -0.0625,
+ "pos_z": 117.96875,
+ "stations": "Holub Penal colony"
+ },
+ {
+ "name": "Albisiyatae",
+ "pos_x": 45.75,
+ "pos_y": -157.0625,
+ "pos_z": -77.375,
+ "stations": "Navigator Camp,Vogel Horizons"
+ },
+ {
+ "name": "Alcha",
+ "pos_x": -106.78125,
+ "pos_y": -83.875,
+ "pos_z": -7.0625,
+ "stations": "Boming Hub"
+ },
+ {
+ "name": "Alchera",
+ "pos_x": -103.5625,
+ "pos_y": -139.1875,
+ "pos_z": 50.6875,
+ "stations": "Nordenskiold Horizons,Lopez de Haro Beacon,Shaver Enterprise"
+ },
+ {
+ "name": "Alchita",
+ "pos_x": 36.21875,
+ "pos_y": 29.28125,
+ "pos_z": 14.21875,
+ "stations": "Favier Dock,Ampere Enterprise,Pennington Port,Searfoss City,Galiano Relay,Lonchakov Dock,Roddenberry Bastion,Ballard Landing,Low Terminal,Dupuy de Lome Installation,Schlegel Dock,Coppel Beacon,Arber Enterprise,Edwards Ring,Antonelli Vision"
+ },
+ {
+ "name": "Alcor",
+ "pos_x": -36,
+ "pos_y": 71.78125,
+ "pos_z": -15.125,
+ "stations": "Macdonald Settlement,Fast Landing,Birmingham Dock,Rawn Prospect"
+ },
+ {
+ "name": "Aldebaran",
+ "pos_x": 1.4375,
+ "pos_y": -22.40625,
+ "pos_z": -62.75,
+ "stations": "Fisher's Rest"
+ },
+ {
+ "name": "Alectrona",
+ "pos_x": 14.53125,
+ "pos_y": -52.46875,
+ "pos_z": 2.5625,
+ "stations": "Marley City,Russ City,Le Guin Port,Back Base"
+ },
+ {
+ "name": "Aleumoxii",
+ "pos_x": 35.09375,
+ "pos_y": 80.28125,
+ "pos_z": -73.40625,
+ "stations": "Ferguson City"
+ },
+ {
+ "name": "Aleut",
+ "pos_x": -136.375,
+ "pos_y": -68.40625,
+ "pos_z": 6.78125,
+ "stations": "Beltrami Colony"
+ },
+ {
+ "name": "Aleuta",
+ "pos_x": 136.78125,
+ "pos_y": 63.3125,
+ "pos_z": 65.84375,
+ "stations": "Burgess Dock,Peirce Settlement,Stephenson Dock,Hadamard's Inheritance,Alexandria Hub"
+ },
+ {
+ "name": "Aleutes",
+ "pos_x": 4.5625,
+ "pos_y": 148.09375,
+ "pos_z": -54.25,
+ "stations": "Buffett Enterprise,Cunningham Landing,Ziemianski Beacon"
+ },
+ {
+ "name": "Alexandrinus",
+ "pos_x": -82.9375,
+ "pos_y": -13.53125,
+ "pos_z": -63.8125,
+ "stations": "Cabo Roig,Khrenov Hub,Evangelisti Reformatory"
+ },
+ {
+ "name": "Alexis Centauri",
+ "pos_x": -41.15625,
+ "pos_y": 23.4375,
+ "pos_z": -108.25,
+ "stations": "Bale,Rosy,King Town"
+ },
+ {
+ "name": "Alfheim",
+ "pos_x": 26.96875,
+ "pos_y": 94.9375,
+ "pos_z": -22.875,
+ "stations": "Gernsback Orbital,Grant Terminal,Panshin Enterprise,Ahmed Point"
+ },
+ {
+ "name": "Algieba",
+ "pos_x": 45.0625,
+ "pos_y": 106.78125,
+ "pos_z": -59.09375,
+ "stations": "Olivas Station,Hart Hub,Kovalevsky Prospect"
+ },
+ {
+ "name": "Algorab",
+ "pos_x": 54.1875,
+ "pos_y": 62.3125,
+ "pos_z": 26.90625,
+ "stations": "O'Leary Terminal,Nicolet Beacon,Stjepan Seljan Stop,Rae Enterprise,Rasmussen Base,Fremion Dock,Tiptree Dock,Forbes Terminal,Dietz Hub,H. G. Wells Enterprise,Kelleam's Claim,Utley Arsenal"
+ },
+ {
+ "name": "Algreit",
+ "pos_x": -8.3125,
+ "pos_y": -11.78125,
+ "pos_z": 53.28125,
+ "stations": "Smith Exchange,Rothman Orbital,Blaschke Orbital"
+ },
+ {
+ "name": "Alhena",
+ "pos_x": 32.0625,
+ "pos_y": 9.59375,
+ "pos_z": -104.0625,
+ "stations": "Bell Terminal,Clough Installation"
+ },
+ {
+ "name": "Alicarl",
+ "pos_x": 33.28125,
+ "pos_y": 95.375,
+ "pos_z": 3.03125,
+ "stations": "Birdseye Port,Parise Ring,Sellings Beacon,Roberts Relay,Shaw Horizons"
+ },
+ {
+ "name": "Alicha",
+ "pos_x": -86.625,
+ "pos_y": 53.09375,
+ "pos_z": 97.53125,
+ "stations": "Davidson Camp,Cabral Beacon"
+ },
+ {
+ "name": "Alichi",
+ "pos_x": -9.4375,
+ "pos_y": 37.15625,
+ "pos_z": 147.3125,
+ "stations": "Lambert Freeport"
+ },
+ {
+ "name": "Alichs",
+ "pos_x": 37.53125,
+ "pos_y": -144.9375,
+ "pos_z": 87.46875,
+ "stations": "Hoerner Point,Bouvard Platform"
+ },
+ {
+ "name": "Alici",
+ "pos_x": -86.84375,
+ "pos_y": -87.96875,
+ "pos_z": 56.84375,
+ "stations": "de Andrade Dock,Fowler Relay"
+ },
+ {
+ "name": "Alignak",
+ "pos_x": -75.03125,
+ "pos_y": 54.53125,
+ "pos_z": 6.75,
+ "stations": "Piserchia Port,Hilbert Gateway,Morrison Vision,Vercors Landing"
+ },
+ {
+ "name": "Alina",
+ "pos_x": 152.53125,
+ "pos_y": -90.78125,
+ "pos_z": -20.875,
+ "stations": "Vogel Station,Koolhaas Vision,Bingzhen Platform"
+ },
+ {
+ "name": "Aling",
+ "pos_x": 19.25,
+ "pos_y": -162.65625,
+ "pos_z": 38,
+ "stations": "Maitz Port,Longomontanus Station,Hartmann Beacon,Brothers Bastion,Cannon Vision"
+ },
+ {
+ "name": "Alino",
+ "pos_x": -0.40625,
+ "pos_y": -33.15625,
+ "pos_z": 87.71875,
+ "stations": "Hadfield Orbital,Haller Depot,Rorschach Enterprise,Lethem Vision,Minkowski Installation"
+ },
+ {
+ "name": "Alint Hozho",
+ "pos_x": 36.3125,
+ "pos_y": -129.625,
+ "pos_z": 134.0625,
+ "stations": "Dixon Hub,Biruni Hub"
+ },
+ {
+ "name": "Alintun",
+ "pos_x": -78.9375,
+ "pos_y": 110.8125,
+ "pos_z": -36.0625,
+ "stations": "Scortia Market,Madsen Arsenal"
+ },
+ {
+ "name": "Alioth",
+ "pos_x": -33.65625,
+ "pos_y": 72.46875,
+ "pos_z": -20.65625,
+ "stations": "Turner Metallics Inc,Laird Dock,Aachen Town,Irkutsk,Mallory Settlement,Golden Gate,Donaldson,Gotham Park,Melbourne Park,Desargues Beacon,Diophantus Works"
+ },
+ {
+ "name": "Alit",
+ "pos_x": 39.84375,
+ "pos_y": -78.5625,
+ "pos_z": -35.96875,
+ "stations": "Albitzky Market,Rittenhouse Dock,Bullialdus Terminal,Bond Port,Webb Port,Morrow Station,Inoda's Inheritance,Otomo Port,Hodgson Keep,Rosse Terminal"
+ },
+ {
+ "name": "Alkabalavs",
+ "pos_x": 104.46875,
+ "pos_y": -117.53125,
+ "pos_z": -47,
+ "stations": "Folland Ring,Aitken Hub,Armero Terminal,Bunch Point,Spassky Beacon"
+ },
+ {
+ "name": "Alkadjar",
+ "pos_x": 34.75,
+ "pos_y": -17.1875,
+ "pos_z": 164.28125,
+ "stations": "Carpenter Point,Hassanein Refinery,Bugrov's Folly"
+ },
+ {
+ "name": "Alkaid",
+ "pos_x": -42.71875,
+ "pos_y": 94.46875,
+ "pos_z": -7.34375,
+ "stations": "Matthewson High,Camus Orbiter,Maxwell City,Pelliot Gateway,Hume Relay,Hassanein Port,Watson Relay,Nagata Orbital,Kent Reach,Russell Vision,Mourelle's Claim"
+ },
+ {
+ "name": "Alkalurops",
+ "pos_x": -58.6875,
+ "pos_y": 100.21875,
+ "pos_z": 33.84375,
+ "stations": "Macquorn Rankine Station,Brunton Hub"
+ },
+ {
+ "name": "Alkes",
+ "pos_x": 126.78125,
+ "pos_y": 96.21875,
+ "pos_z": -0.34375,
+ "stations": "Burkin Barracks,Grabe Installation"
+ },
+ {
+ "name": "Alkh",
+ "pos_x": 17.125,
+ "pos_y": 76.90625,
+ "pos_z": -61.3125,
+ "stations": "Altman Laboratory,Jones Gateway,Jemison Orbital,Fairbairn Prospect"
+ },
+ {
+ "name": "Alklhaluana",
+ "pos_x": -10.46875,
+ "pos_y": 61.65625,
+ "pos_z": -150.28125,
+ "stations": "Russell Platform,Payson Orbital,Larson Depot"
+ },
+ {
+ "name": "Alkondra",
+ "pos_x": 0.03125,
+ "pos_y": 17.5625,
+ "pos_z": -144.53125,
+ "stations": "Carson City,Zholobov City,Cavendish Settlement,Chiang Landing,Drexler Survey"
+ },
+ {
+ "name": "Alkondu",
+ "pos_x": 90.75,
+ "pos_y": -21.65625,
+ "pos_z": -174.125,
+ "stations": "Pond Outpost"
+ },
+ {
+ "name": "Alkondul",
+ "pos_x": -79.15625,
+ "pos_y": 58.15625,
+ "pos_z": -144.6875,
+ "stations": "Riemann City"
+ },
+ {
+ "name": "Alkundan",
+ "pos_x": -52.125,
+ "pos_y": 41.78125,
+ "pos_z": 122.5,
+ "stations": "Fontenay Landing,Wollheim Dock"
+ },
+ {
+ "name": "Alkunn",
+ "pos_x": 58.84375,
+ "pos_y": 126.5625,
+ "pos_z": 32.59375,
+ "stations": "Akers Dock"
+ },
+ {
+ "name": "Alkupai",
+ "pos_x": -80.875,
+ "pos_y": 105.65625,
+ "pos_z": -71.15625,
+ "stations": "Stott Orbital,Crouch Landing,Kozeyev Depot,Terry Hub"
+ },
+ {
+ "name": "Alkuylkanam",
+ "pos_x": -58.59375,
+ "pos_y": 82.625,
+ "pos_z": 65.84375,
+ "stations": "Miletus Terminal,Lovelace Port,Deb Hub,Sullivan Enterprise,Strekalov Terminal,Ciferri Port,Zoline Settlement,Gurney Hub,Forstchen Depot,Baydukov Keep"
+ },
+ {
+ "name": "Alkuylkano",
+ "pos_x": 144.4375,
+ "pos_y": 7.15625,
+ "pos_z": 18.875,
+ "stations": "Bowen Colony,Ryumin Orbital,Frick Station,Sharp Installation,Langsdorff Survey"
+ },
+ {
+ "name": "Alkuyuma",
+ "pos_x": 77.59375,
+ "pos_y": 5.6875,
+ "pos_z": -34.96875,
+ "stations": "Khan Point,Brunton Point"
+ },
+ {
+ "name": "Allechs",
+ "pos_x": 121.65625,
+ "pos_y": -5.59375,
+ "pos_z": 58.625,
+ "stations": "Mitchell Depot,Stasheff Works"
+ },
+ {
+ "name": "Allentiac",
+ "pos_x": 58.09375,
+ "pos_y": -186.0625,
+ "pos_z": 11.78125,
+ "stations": "Safdie Beacon"
+ },
+ {
+ "name": "Alles",
+ "pos_x": 169.625,
+ "pos_y": -56.09375,
+ "pos_z": 27.96875,
+ "stations": "Tank Holdings,Perrine Estate,Rangarajan's Folly"
+ },
+ {
+ "name": "Allo",
+ "pos_x": -166.40625,
+ "pos_y": -23.875,
+ "pos_z": -38.375,
+ "stations": "Crick Landing,Lopez-Alegria Orbital,Pavlov Orbital,Clark's Progress"
+ },
+ {
+ "name": "Allobo",
+ "pos_x": 89.3125,
+ "pos_y": -7.65625,
+ "pos_z": 60.4375,
+ "stations": "Tavernier Landing,Leckie Colony"
+ },
+ {
+ "name": "Allobog",
+ "pos_x": -92.9375,
+ "pos_y": -38.0625,
+ "pos_z": 121.03125,
+ "stations": "McHugh Station,Alexander Enterprise,Andersson Depot,Jordan Reach"
+ },
+ {
+ "name": "Allocadros",
+ "pos_x": 44.78125,
+ "pos_y": 11.59375,
+ "pos_z": 69.875,
+ "stations": "Stewart Orbital"
+ },
+ {
+ "name": "Allocassa",
+ "pos_x": 102.875,
+ "pos_y": 58.25,
+ "pos_z": -106.15625,
+ "stations": "Tilman Escape,Matthews Vision,Rucker Prospect"
+ },
+ {
+ "name": "Allovana",
+ "pos_x": 125.25,
+ "pos_y": -102.53125,
+ "pos_z": 40.53125,
+ "stations": "Narlikar's Claim,Hussenot Orbital,de Kamp Hub"
+ },
+ {
+ "name": "Allovaste",
+ "pos_x": 96.5,
+ "pos_y": -64.78125,
+ "pos_z": -52.96875,
+ "stations": "Lewitt Port,Hardy Enterprise,Lorrah Horizons"
+ },
+ {
+ "name": "Allowa",
+ "pos_x": -67.125,
+ "pos_y": 21.21875,
+ "pos_z": 80.40625,
+ "stations": "Moore Ring,Cormack Horizons,Gaspar de Portola Terminal,Acton Station"
+ },
+ {
+ "name": "Allowair",
+ "pos_x": -80.75,
+ "pos_y": -3.5,
+ "pos_z": 55.5625,
+ "stations": "Underwood Survey,Skripochka Survey,Nachtigal Survey,Gernhardt Landing"
+ },
+ {
+ "name": "Allowini",
+ "pos_x": -43.34375,
+ "pos_y": 4.03125,
+ "pos_z": -126.6875,
+ "stations": "Chiao Landing,Libby Terminal,Farkas Prospect"
+ },
+ {
+ "name": "Almad",
+ "pos_x": 97.875,
+ "pos_y": -116.75,
+ "pos_z": -31.96875,
+ "stations": "Uto Horizons,Heinkel City,McCool Point"
+ },
+ {
+ "name": "Almagest",
+ "pos_x": -274.65625,
+ "pos_y": -10.8125,
+ "pos_z": -271.34375,
+ "stations": "Sirius Reach,Attenborough Terminal,Pride's Landing"
+ },
+ {
+ "name": "Almana",
+ "pos_x": -95.625,
+ "pos_y": 71,
+ "pos_z": 55.125,
+ "stations": "Robinson Port"
+ },
+ {
+ "name": "Almar",
+ "pos_x": 146.3125,
+ "pos_y": -149.03125,
+ "pos_z": -84.96875,
+ "stations": "Henderson Colony"
+ },
+ {
+ "name": "Almas",
+ "pos_x": 30.84375,
+ "pos_y": -117.65625,
+ "pos_z": 79.6875,
+ "stations": "McCrea Vision,Vakhmistrov Barracks,Porubcan Prospect,Polya Laboratory,Schroter Market,von Zach Orbital"
+ },
+ {
+ "name": "Almasci",
+ "pos_x": 200.28125,
+ "pos_y": -177.15625,
+ "pos_z": 21.53125,
+ "stations": "Stiles Base,Dashiell Stop"
+ },
+ {
+ "name": "Almudja",
+ "pos_x": 70.59375,
+ "pos_y": 55.375,
+ "pos_z": -16.625,
+ "stations": "Meikle Dock,Cadamosto Silo"
+ },
+ {
+ "name": "Almudjara",
+ "pos_x": 40.15625,
+ "pos_y": -194.90625,
+ "pos_z": -6.96875,
+ "stations": "Cerulli Station,Bode City,Richer Ring,Pond Depot,Theodorsen Stop"
+ },
+ {
+ "name": "Almudji",
+ "pos_x": 104.125,
+ "pos_y": -67.25,
+ "pos_z": 58.65625,
+ "stations": "Corbusier Landing,Thierree Dock"
+ },
+ {
+ "name": "Almudjing",
+ "pos_x": 8.84375,
+ "pos_y": 81.46875,
+ "pos_z": 116.375,
+ "stations": "Brandenstein Outpost,Sherrington Reach"
+ },
+ {
+ "name": "Alnair",
+ "pos_x": 10.40625,
+ "pos_y": -80.75,
+ "pos_z": 59.78125,
+ "stations": "King Prospect,Cowper Freeport,Brooks Landing"
+ },
+ {
+ "name": "Alourok",
+ "pos_x": -128.375,
+ "pos_y": -2.84375,
+ "pos_z": 130.25,
+ "stations": "Pangborn Reach"
+ },
+ {
+ "name": "Alouroua",
+ "pos_x": 41.6875,
+ "pos_y": -208.46875,
+ "pos_z": 6.90625,
+ "stations": "Brin Bastion,Nomura Settlement,Hewish Survey"
+ },
+ {
+ "name": "Alourovices",
+ "pos_x": 172.53125,
+ "pos_y": -184.125,
+ "pos_z": 87.03125,
+ "stations": "Opik Orbital,Vuia Dock,Arkhangelsky City,Harding Depot"
+ },
+ {
+ "name": "Alowali",
+ "pos_x": 139.9375,
+ "pos_y": 60.6875,
+ "pos_z": 39.75,
+ "stations": "Read Mines"
+ },
+ {
+ "name": "Alowis",
+ "pos_x": -28.625,
+ "pos_y": 24.875,
+ "pos_z": 116.34375,
+ "stations": "Killough Beacon,Song Refinery"
+ },
+ {
+ "name": "Alpha Caeli",
+ "pos_x": 45.21875,
+ "pos_y": -43.34375,
+ "pos_z": -20.125,
+ "stations": "Heyerdahl Hub,Eckford Port,Becquerel's Folly,Alexeyev Ring,Dall's Inheritance,Shosuke Hub,Leonov Depot,Pohl Laboratory,Nobel Horizons"
+ },
+ {
+ "name": "Alpha Centauri",
+ "pos_x": 3.03125,
+ "pos_y": -0.09375,
+ "pos_z": 3.15625,
+ "stations": "al-Din Prospect,Hutton Orbital"
+ },
+ {
+ "name": "Alpha Chamaelontis",
+ "pos_x": 55.65625,
+ "pos_y": -23.75,
+ "pos_z": 20.21875,
+ "stations": "Rorschach Silo,Vernadsky Colony"
+ },
+ {
+ "name": "Alpha Fornacis",
+ "pos_x": 16.9375,
+ "pos_y": -39.625,
+ "pos_z": -17.3125,
+ "stations": "Clement Station,Banks Vision,Wandrei Gateway,Doi City,Helms Hub,McIntyre Lab,Kidman Works,Blenkinsop Penal colony,Bennett Port"
+ },
+ {
+ "name": "Alpha Octantis",
+ "pos_x": 81.71875,
+ "pos_y": -79.5,
+ "pos_z": 84.625,
+ "stations": "Swift Orbital"
+ },
+ {
+ "name": "Alpha Reticuli",
+ "pos_x": 120.46875,
+ "pos_y": -107.375,
+ "pos_z": 8.65625,
+ "stations": "Webb Mines"
+ },
+ {
+ "name": "Alpha Tucanae",
+ "pos_x": 65.875,
+ "pos_y": -149.5,
+ "pos_z": 114.90625,
+ "stations": "Alexandria Terminal,Peebles Port,van der Riet Woolley Palace,Tisserand Orbital,Waldeck's Claim,Zamyatin Gateway,Chretien Oudemans Settlement,Cuffey Hub,Barnard Orbital,Calatrava Terminal,Bradfield Dock,Lemonnier Station,Carsono Port,Gabriel Depot,Kotelnikov Refinery"
+ },
+ {
+ "name": "Alpha Volantis",
+ "pos_x": 118.59375,
+ "pos_y": -28.28125,
+ "pos_z": 27.1875,
+ "stations": "Oramus Vista,Reid Refinery"
+ },
+ {
+ "name": "Alpheus",
+ "pos_x": 2.03125,
+ "pos_y": -56.375,
+ "pos_z": 56.21875,
+ "stations": "Walker Port,Bardeen Settlement,Kimberlin Camp"
+ },
+ {
+ "name": "Alrai",
+ "pos_x": -38.71875,
+ "pos_y": 12.3125,
+ "pos_z": -21.625,
+ "stations": "Bounds Hub"
+ },
+ {
+ "name": "Alrai Sector DL-Y d156",
+ "pos_x": -55.9375,
+ "pos_y": 41.875,
+ "pos_z": 127.9375,
+ "stations": "Peary Penal colony,Howard's Progress"
+ },
+ {
+ "name": "Alrai Sector HR-W c1-13",
+ "pos_x": -93.28125,
+ "pos_y": 2.5,
+ "pos_z": 87.25,
+ "stations": "Walheim Enterprise"
+ },
+ {
+ "name": "Alrai Sector JC-V c2-28",
+ "pos_x": -81.75,
+ "pos_y": 22.53125,
+ "pos_z": 105.75,
+ "stations": "King Works,Fermat Beacon,Macarthur Depot,Mach Observatory"
+ },
+ {
+ "name": "Alrai Sector KH-V b2-7",
+ "pos_x": -41.34375,
+ "pos_y": 1.46875,
+ "pos_z": 61.6875,
+ "stations": "Mercy's Hammer"
+ },
+ {
+ "name": "Alrai Sector KN-S b4-4",
+ "pos_x": -34.96875,
+ "pos_y": 77.625,
+ "pos_z": 101.59375,
+ "stations": "Mother of Redemption"
+ },
+ {
+ "name": "Alrai Sector PT-R b4-5",
+ "pos_x": -85.09375,
+ "pos_y": 12.15625,
+ "pos_z": 105.65625,
+ "stations": "Gaspar de Lemos Survey,Chasles Beacon,Kotzebue's Progress"
+ },
+ {
+ "name": "Alrai Sector VZ-P b5-2",
+ "pos_x": -48.59375,
+ "pos_y": 0.53125,
+ "pos_z": 123.8125,
+ "stations": "Cousteau Depot,Plexico Laboratory,Macedo Reach"
+ },
+ {
+ "name": "Alrisha",
+ "pos_x": -34.875,
+ "pos_y": -123.46875,
+ "pos_z": -78.84375,
+ "stations": "Fearn Survey"
+ },
+ {
+ "name": "ALS 16791",
+ "pos_x": 29.125,
+ "pos_y": -52.03125,
+ "pos_z": 86.6875,
+ "stations": "Malerba Terminal,Hornblower Terminal,Ivanishin Hub"
+ },
+ {
+ "name": "Altair",
+ "pos_x": -12.3125,
+ "pos_y": -2.75,
+ "pos_z": 11,
+ "stations": "Solo Orbiter,Tereshkova Dock,Grandin Gateway,Jones Vision"
+ },
+ {
+ "name": "Altais",
+ "pos_x": -88.625,
+ "pos_y": 38.09375,
+ "pos_z": -13.65625,
+ "stations": "Swanson Plant,Fairbairn Holdings"
+ },
+ {
+ "name": "Altani",
+ "pos_x": 114.125,
+ "pos_y": -115.71875,
+ "pos_z": -62.03125,
+ "stations": "Rubin Port,Bond Dock,Hartmann Ring,Burroughs' Progress,Tanaka Refinery,Bryant Vision"
+ },
+ {
+ "name": "Altauluwa",
+ "pos_x": 160.25,
+ "pos_y": -77.09375,
+ "pos_z": 76.84375,
+ "stations": "Lockyer Hub,Euthymenes Vision,Vlaicu Ring,Bliss Port"
+ },
+ {
+ "name": "Aluremowo",
+ "pos_x": 66.03125,
+ "pos_y": -146.09375,
+ "pos_z": 120.15625,
+ "stations": "Robert Stop,Schoenherr Penal colony"
+ },
+ {
+ "name": "Aluriates",
+ "pos_x": 144.59375,
+ "pos_y": -176.1875,
+ "pos_z": 50.71875,
+ "stations": "Elmore City,Hirn Dock,Maughmer Ring,Adams Orbital,Montanari Dock,Fraknoi Terminal,Wnuk-Lipinski Stop,Auer Observatory,Constantine Landing,Saha Orbital,Deutsch Landing,Dawes Reach,Barton Arsenal,Roemer Hub,Nicholson Hub"
+ },
+ {
+ "name": "Aluroua",
+ "pos_x": 26.90625,
+ "pos_y": -73.96875,
+ "pos_z": 154.84375,
+ "stations": "Wilcutt Base"
+ },
+ {
+ "name": "Aluru",
+ "pos_x": -41.09375,
+ "pos_y": -34.625,
+ "pos_z": -129.1875,
+ "stations": "Foden Base,Rasch's Progress"
+ },
+ {
+ "name": "Alya",
+ "pos_x": -77.28125,
+ "pos_y": -57.4375,
+ "pos_z": 30.53125,
+ "stations": "Malaspina Gateway,Rayhan al-Biruni Ring,Zamka Escape,Zamyatin Enterprise"
+ },
+ {
+ "name": "Alyuta",
+ "pos_x": 5.625,
+ "pos_y": -171.21875,
+ "pos_z": -28.84375,
+ "stations": "Bahcall Vision,Whitford Installation,Dashiell Lab"
+ },
+ {
+ "name": "Alyuto",
+ "pos_x": -47.25,
+ "pos_y": 71,
+ "pos_z": -15.84375,
+ "stations": "Levinson Vision"
+ },
+ {
+ "name": "Amahibuhcha",
+ "pos_x": 95.65625,
+ "pos_y": -120.5625,
+ "pos_z": 102.375,
+ "stations": "Wallerstein Prospect,Foster Terminal,Pond Station"
+ },
+ {
+ "name": "Amahine",
+ "pos_x": 22.65625,
+ "pos_y": 105.90625,
+ "pos_z": -87.875,
+ "stations": "Lloyd Dock"
+ },
+ {
+ "name": "Amahinohara",
+ "pos_x": 83.15625,
+ "pos_y": -9.96875,
+ "pos_z": -141,
+ "stations": "Roentgen Landing,Herschel Prospect,vo Horizons,Nansen Bastion,Preuss Base"
+ },
+ {
+ "name": "Amahu",
+ "pos_x": -30.40625,
+ "pos_y": 39.4375,
+ "pos_z": 77.3125,
+ "stations": "Yaping Enterprise,Burbank Hub,Kondratyev Orbital,Clifford Station,Bogdanov's Folly,Barsanti Orbital,Grissom Enterprise,Musabayev Port,Alexandria Gateway,Marshall Dock,Semeonis' Inheritance,Hieb Keep,Weinbaum's Inheritance"
+ },
+ {
+ "name": "Amahuaca",
+ "pos_x": -16.875,
+ "pos_y": -181.84375,
+ "pos_z": 66.78125,
+ "stations": "Arend Station,Suntzeff Hub,Kandrup Gateway"
+ },
+ {
+ "name": "Amahumasi",
+ "pos_x": 155.375,
+ "pos_y": -40.59375,
+ "pos_z": 66.0625,
+ "stations": "Winthrop Terminal,van Houten City,Goldreich City,Tudela Silo,Key Plant"
+ },
+ {
+ "name": "Amahutii",
+ "pos_x": -111.03125,
+ "pos_y": -117.46875,
+ "pos_z": 37.1875,
+ "stations": "Oleskiw Colony"
+ },
+ {
+ "name": "Amairii",
+ "pos_x": -92.375,
+ "pos_y": 10.78125,
+ "pos_z": 61.96875,
+ "stations": "Frimout Relay,Guest Terminal,Valdes Base"
+ },
+ {
+ "name": "Amait",
+ "pos_x": 27.15625,
+ "pos_y": -13.6875,
+ "pos_z": -67.5,
+ "stations": "Lopez de Villalobos Prospect,Oramus Legacy"
+ },
+ {
+ "name": "Amaitsa",
+ "pos_x": -78.71875,
+ "pos_y": -65.15625,
+ "pos_z": 85.625,
+ "stations": "Narvaez Dock,Hutchinson Colony,McCarthy Dock,Lopez de Haro Point,Spedding Terminal"
+ },
+ {
+ "name": "Amala",
+ "pos_x": -49.0625,
+ "pos_y": 69.9375,
+ "pos_z": 84.34375,
+ "stations": "Redi Relay"
+ },
+ {
+ "name": "Amalangkan",
+ "pos_x": 66.3125,
+ "pos_y": 24.1875,
+ "pos_z": 43.3125,
+ "stations": "Doi Landing"
+ },
+ {
+ "name": "Aman Zaihe",
+ "pos_x": 105.9375,
+ "pos_y": -255.9375,
+ "pos_z": 17.28125,
+ "stations": "Carroll Colony,Francisco de Almeida Point,Abetti Colony"
+ },
+ {
+ "name": "Amanaye",
+ "pos_x": -107.125,
+ "pos_y": -121.125,
+ "pos_z": -21.75,
+ "stations": "Mieville Port,Hawke Prospect,Due Gateway,Garden Legacy,Margulies Terminal,Wilkes' Claim"
+ },
+ {
+ "name": "Amanellos",
+ "pos_x": 116.96875,
+ "pos_y": -32.375,
+ "pos_z": 116.71875,
+ "stations": "Barnes Retreat"
+ },
+ {
+ "name": "Amaneque",
+ "pos_x": 67.46875,
+ "pos_y": 6.9375,
+ "pos_z": 87.09375,
+ "stations": "Gilliland Colony"
+ },
+ {
+ "name": "Amang Tzu",
+ "pos_x": -58.15625,
+ "pos_y": 119,
+ "pos_z": 20.71875,
+ "stations": "Jahn Settlement,Afanasyev City,Walter Horizons,Ocampo Bastion,Fulton Survey"
+ },
+ {
+ "name": "Amanii",
+ "pos_x": -81,
+ "pos_y": -64.8125,
+ "pos_z": 120,
+ "stations": "Phillifent Settlement,Dietz Colony"
+ },
+ {
+ "name": "Amantai",
+ "pos_x": -93.1875,
+ "pos_y": 19.84375,
+ "pos_z": -119.40625,
+ "stations": "Cixin Terminal,Holland Vista,Burgess Installation,Fremion Terminal"
+ },
+ {
+ "name": "Amaragua",
+ "pos_x": -93.8125,
+ "pos_y": -20.34375,
+ "pos_z": -81.75,
+ "stations": "Harris Hub,Bean Gateway,Edgeworth Hub,Heaviside Keep"
+ },
+ {
+ "name": "Amarak",
+ "pos_x": -68.4375,
+ "pos_y": 26,
+ "pos_z": 26.46875,
+ "stations": "Vela Port,Curie Silo,Werner von Siemens Vision,Marconi Enterprise"
+ },
+ {
+ "name": "Amarakaeri",
+ "pos_x": -4.625,
+ "pos_y": 37,
+ "pos_z": 135.65625,
+ "stations": "Stephenson Depot"
+ },
+ {
+ "name": "Amaram",
+ "pos_x": -81.15625,
+ "pos_y": 77.09375,
+ "pos_z": 127.28125,
+ "stations": "Grzimek Platform,Laird Camp,Julian's Progress"
+ },
+ {
+ "name": "Amariba",
+ "pos_x": -123.25,
+ "pos_y": 43.1875,
+ "pos_z": 80.5625,
+ "stations": "Newcomen Gateway,Dobrovolski Dock,Apt Hub,Fidalgo Silo"
+ },
+ {
+ "name": "Amarigeni",
+ "pos_x": 100.625,
+ "pos_y": -93.21875,
+ "pos_z": 72.8125,
+ "stations": "Bowen Platform,Walotsky Port"
+ },
+ {
+ "name": "Amarishvaru",
+ "pos_x": 102.96875,
+ "pos_y": -16.5,
+ "pos_z": -44.625,
+ "stations": "Theodor Winnecke Settlement"
+ },
+ {
+ "name": "Amaterasu",
+ "pos_x": 51.21875,
+ "pos_y": -15.28125,
+ "pos_z": -14.5,
+ "stations": "Ramsay Port,Sacco Refinery"
+ },
+ {
+ "name": "Amatsuboshi",
+ "pos_x": -9516.03125,
+ "pos_y": -908.25,
+ "pos_z": 19802.71875,
+ "stations": "Hayabusa Landing"
+ },
+ {
+ "name": "Amatyerus",
+ "pos_x": -93.375,
+ "pos_y": -123.875,
+ "pos_z": -77.28125,
+ "stations": "Bao Hub,Bryusov Settlement"
+ },
+ {
+ "name": "Amaucae",
+ "pos_x": 64.84375,
+ "pos_y": -83.28125,
+ "pos_z": -71.90625,
+ "stations": "Massimino Hub,Cummings Works,Dobzhansky Landing"
+ },
+ {
+ "name": "Amauci",
+ "pos_x": 67.9375,
+ "pos_y": -129.03125,
+ "pos_z": -91.6875,
+ "stations": "Eggen Ring,Canty Gateway,Davis Penal colony,Quinn Vision"
+ },
+ {
+ "name": "Amaunet",
+ "pos_x": -35.28125,
+ "pos_y": -58.40625,
+ "pos_z": -101.375,
+ "stations": "North Dock"
+ },
+ {
+ "name": "Ambare",
+ "pos_x": -44.53125,
+ "pos_y": -164.875,
+ "pos_z": -41.84375,
+ "stations": "Kojima Enterprise,Hamuy Station,Kopal Dock,Reid Landing"
+ },
+ {
+ "name": "Amber",
+ "pos_x": -36.1875,
+ "pos_y": 77.3125,
+ "pos_z": -32.53125,
+ "stations": "Felice Plant,Smith Landing,Hahn's Folly"
+ },
+ {
+ "name": "Ambiabar",
+ "pos_x": 82,
+ "pos_y": -151.5,
+ "pos_z": 30.1875,
+ "stations": "Mizuno Settlement,Rosenberg Hub"
+ },
+ {
+ "name": "Ambians",
+ "pos_x": 51.25,
+ "pos_y": 80.78125,
+ "pos_z": -135.8125,
+ "stations": "Maine Camp,Vaez de Torres Plant"
+ },
+ {
+ "name": "Ambibarii",
+ "pos_x": -124.46875,
+ "pos_y": -105.09375,
+ "pos_z": -22.09375,
+ "stations": "Shelley Point,Crowley Terminal,West Vision,Gunn Station,Steinmuller Camp"
+ },
+ {
+ "name": "Ambibates",
+ "pos_x": 25.59375,
+ "pos_y": -39.28125,
+ "pos_z": 52.15625,
+ "stations": "Stephan Terminal"
+ },
+ {
+ "name": "Ambibeb",
+ "pos_x": 94.34375,
+ "pos_y": -11.75,
+ "pos_z": -43.9375,
+ "stations": "Pontes Platform,Caidin's Folly,Yegorov Depot"
+ },
+ {
+ "name": "Ambibio",
+ "pos_x": 128.875,
+ "pos_y": 91.75,
+ "pos_z": 7.65625,
+ "stations": "Cseszneky Ring,Mastracchio Hub,Rushd Port,Samuda's Progress,Brunton Enterprise"
+ },
+ {
+ "name": "Ambika",
+ "pos_x": 90.75,
+ "pos_y": 140.25,
+ "pos_z": 69.375,
+ "stations": "Goulart City,Russ Dock,Grimwood Holdings,Bayley Holdings"
+ },
+ {
+ "name": "Ambikul",
+ "pos_x": -59.90625,
+ "pos_y": -60.75,
+ "pos_z": -94.9375,
+ "stations": "Tepper Ring,Kingsmill Enterprise,Benz Settlement,Blaschke Prospect,Zindell Enterprise"
+ },
+ {
+ "name": "Ambiliates",
+ "pos_x": 97.03125,
+ "pos_y": -97.5,
+ "pos_z": 81.96875,
+ "stations": "Hanke-Woods Terminal,Crown Penal colony,Addams Vision,Henry Port,Denton Prospect"
+ },
+ {
+ "name": "Ambiri",
+ "pos_x": -43.90625,
+ "pos_y": 22.34375,
+ "pos_z": 71.96875,
+ "stations": "Chasles Orbital,Longyear's Inheritance,Eisele Arsenal"
+ },
+ {
+ "name": "Ambirikar",
+ "pos_x": 57.875,
+ "pos_y": -95.125,
+ "pos_z": -53.25,
+ "stations": "Wallis Landing"
+ },
+ {
+ "name": "Ambishas",
+ "pos_x": -40.375,
+ "pos_y": 53.96875,
+ "pos_z": 111.78125,
+ "stations": "Wheeler Point,Covey Beacon,Mukai Enterprise"
+ },
+ {
+ "name": "Ambivas",
+ "pos_x": 6.65625,
+ "pos_y": -100.15625,
+ "pos_z": 50.59375,
+ "stations": "Baillaud Port,Yize Colony"
+ },
+ {
+ "name": "Ambo",
+ "pos_x": 134.5,
+ "pos_y": -116.5625,
+ "pos_z": 48.40625,
+ "stations": "Corbusier City,Hooker Terminal,Carlisle Base,Andrews Barracks,Gulyaev Horizons"
+ },
+ {
+ "name": "Amemait",
+ "pos_x": 154.25,
+ "pos_y": -17.34375,
+ "pos_z": -77.875,
+ "stations": "Guerrero Relay,Asprin Beacon"
+ },
+ {
+ "name": "Amemakarna",
+ "pos_x": -24.125,
+ "pos_y": 55.75,
+ "pos_z": -142.0625,
+ "stations": "Born Hub"
+ },
+ {
+ "name": "Amemasa",
+ "pos_x": 28.59375,
+ "pos_y": -44.3125,
+ "pos_z": 115.84375,
+ "stations": "Clark Mine,Bombelli Landing,Sharman Dock"
+ },
+ {
+ "name": "Amementi",
+ "pos_x": 62.96875,
+ "pos_y": 27.6875,
+ "pos_z": 107.15625,
+ "stations": "Buckey Hub,Baker Forum"
+ },
+ {
+ "name": "Amemets",
+ "pos_x": 87.90625,
+ "pos_y": -81.5625,
+ "pos_z": -30.8125,
+ "stations": "Smith Dock,Benyovszky Horizons"
+ },
+ {
+ "name": "Amen Hozho",
+ "pos_x": -62.21875,
+ "pos_y": 117.65625,
+ "pos_z": -68.71875,
+ "stations": "Starzl Horizons"
+ },
+ {
+ "name": "Amenhit",
+ "pos_x": -17.46875,
+ "pos_y": -99.5,
+ "pos_z": 140.65625,
+ "stations": "Carroll Beacon"
+ },
+ {
+ "name": "Amenhotep",
+ "pos_x": -78.75,
+ "pos_y": -141.90625,
+ "pos_z": 61.78125,
+ "stations": "Coulomb Camp,Celsius Outpost"
+ },
+ {
+ "name": "Amenili",
+ "pos_x": 115.71875,
+ "pos_y": -181.15625,
+ "pos_z": 89.6875,
+ "stations": "Mattingly Vista,Medupe's Folly,Oja Vista,Kingsbury Holdings"
+ },
+ {
+ "name": "Amenni",
+ "pos_x": 53.9375,
+ "pos_y": 44.65625,
+ "pos_z": 56.90625,
+ "stations": "Sabine Arena"
+ },
+ {
+ "name": "Amenniya",
+ "pos_x": -50.25,
+ "pos_y": -43.75,
+ "pos_z": -72.09375,
+ "stations": "Mawson Terminal,Fremont Port,Nixon Port,Paez Prospect"
+ },
+ {
+ "name": "Ameno",
+ "pos_x": 127.71875,
+ "pos_y": -132.71875,
+ "pos_z": 60,
+ "stations": "Vakhmistrov Vision"
+ },
+ {
+ "name": "Amenta",
+ "pos_x": 140.09375,
+ "pos_y": -107.0625,
+ "pos_z": 22.375,
+ "stations": "Ernst Port,Schmidt City,Dilworth Ring,Dozois Base,Bauschinger City,Wafa Gateway,Young City,Okuni Port,Unsold Prospect,Nielsen Beacon,Orbik Ring,Eggen Dock,Tem Hub,Hill Barracks,Westerfeld Port"
+ },
+ {
+ "name": "Amente",
+ "pos_x": 64.1875,
+ "pos_y": -62.8125,
+ "pos_z": 76.90625,
+ "stations": "Womack Enterprise,von Helmont Hub,Evans Station,Ansari Gateway"
+ },
+ {
+ "name": "Amentsura",
+ "pos_x": 24.9375,
+ "pos_y": -179,
+ "pos_z": 88.65625,
+ "stations": "Fox Relay"
+ },
+ {
+ "name": "Amicus",
+ "pos_x": -51.1875,
+ "pos_y": 165.0625,
+ "pos_z": -16.75,
+ "stations": "Mills Colony"
+ },
+ {
+ "name": "Amijangal",
+ "pos_x": 79.25,
+ "pos_y": 37.5625,
+ "pos_z": 56.5625,
+ "stations": "Bushkov Beacon,Gooch Works,Dana Point"
+ },
+ {
+ "name": "Amijara",
+ "pos_x": -38.28125,
+ "pos_y": -21.5,
+ "pos_z": -124.09375,
+ "stations": "Shepherd Outpost,Xiaoguan Terminal"
+ },
+ {
+ "name": "Amis",
+ "pos_x": 20.5,
+ "pos_y": -22.3125,
+ "pos_z": -122.8125,
+ "stations": "Carlisle's Progress,Perga Relay"
+ },
+ {
+ "name": "Amisi",
+ "pos_x": -40.90625,
+ "pos_y": -19.84375,
+ "pos_z": -118.625,
+ "stations": "Sargent Escape,Brooks' Folly,Lysenko Stop"
+ },
+ {
+ "name": "Amit",
+ "pos_x": 0.75,
+ "pos_y": -0.90625,
+ "pos_z": 145.3125,
+ "stations": "Tevis Hangar"
+ },
+ {
+ "name": "Amitabha",
+ "pos_x": 124.09375,
+ "pos_y": 14.25,
+ "pos_z": -146.15625,
+ "stations": "Bulmer Settlement,Ziljak Enterprise,Bailey Vision,Hodgkinson Landing,Gibbs Camp"
+ },
+ {
+ "name": "Amitae",
+ "pos_x": 171.0625,
+ "pos_y": 11.1875,
+ "pos_z": 72.78125,
+ "stations": "Elgin Landing,Jacobi Settlement,Shosuke Vision"
+ },
+ {
+ "name": "Amitrebes",
+ "pos_x": -24.8125,
+ "pos_y": -199.03125,
+ "pos_z": -0.5625,
+ "stations": "Krenkel Oasis,Jackson Colony,Shumil Reach"
+ },
+ {
+ "name": "Amitrimpanu",
+ "pos_x": 19.9375,
+ "pos_y": -111.1875,
+ "pos_z": -91.625,
+ "stations": "Williams Survey,Kubokawa Port"
+ },
+ {
+ "name": "Amitrite",
+ "pos_x": 99.875,
+ "pos_y": -110.34375,
+ "pos_z": -6.25,
+ "stations": "Cook Installation,Pilcher Terminal,Dhawan Port,Ashton Point"
+ },
+ {
+ "name": "Ammaiamh",
+ "pos_x": 20.15625,
+ "pos_y": 48.96875,
+ "pos_z": 146.03125,
+ "stations": "Henslow Platform,Flynn Installation,Gottlob Frege's Claim"
+ },
+ {
+ "name": "Ammapa",
+ "pos_x": -115.25,
+ "pos_y": 58.125,
+ "pos_z": -43.28125,
+ "stations": "Hennen Port,Gwynn Mines,Fischer Terminal,Viktorenko Port,Virts Dock,Cseszneky Terminal,Tyson Orbital,Covey Hub,Vinogradov Hub,Pournelle Vision,Resnick Depot,Reed's Inheritance"
+ },
+ {
+ "name": "Ammapatha",
+ "pos_x": 56.625,
+ "pos_y": -1.4375,
+ "pos_z": -139.53125,
+ "stations": "Komarov Camp,Mayr Beacon"
+ },
+ {
+ "name": "Ammata",
+ "pos_x": 102.1875,
+ "pos_y": 35.9375,
+ "pos_z": -118.625,
+ "stations": "Langsdorff Survey,van Vogt Enterprise,Mitchell Plant"
+ },
+ {
+ "name": "Ammatch",
+ "pos_x": 110.34375,
+ "pos_y": -124.40625,
+ "pos_z": 25.3125,
+ "stations": "Suydam Relay"
+ },
+ {
+ "name": "Ammatis",
+ "pos_x": 16.1875,
+ "pos_y": 22.28125,
+ "pos_z": 108.375,
+ "stations": "Barr Landing,Zillig Orbital,Navigator Dock,Monge Hub,Kafka Market,Irens Relay,Murphy City,Hippalus Terminal,Houtman Station,Fairbairn Vision"
+ },
+ {
+ "name": "Amo",
+ "pos_x": 3.65625,
+ "pos_y": -80.8125,
+ "pos_z": 42.875,
+ "stations": "Kojima Refinery,Boyajian Holdings"
+ },
+ {
+ "name": "Amphiloci",
+ "pos_x": -102.75,
+ "pos_y": -41.03125,
+ "pos_z": -88.21875,
+ "stations": "Blair Colony,Reed Plant,Crown Prospect"
+ },
+ {
+ "name": "Amphisatsu",
+ "pos_x": 67.375,
+ "pos_y": -72.3125,
+ "pos_z": 21.78125,
+ "stations": "Goldberg Orbital,Watanabe Terminal,Karachkina Hub,Osterbrock Terminal,Amundsen Vision,Smirnova Station,Brashear Landing,Giacobini Plant"
+ },
+ {
+ "name": "Amsitia",
+ "pos_x": 19.4375,
+ "pos_y": -170.53125,
+ "pos_z": -0.28125,
+ "stations": "Firsoff Gateway,Pimi Vision,Ziegel Port,Canty Laboratory,Shklovsky Base"
+ },
+ {
+ "name": "Amun",
+ "pos_x": 51.5625,
+ "pos_y": 32.1875,
+ "pos_z": -27.125,
+ "stations": "Moresby Dock,Baker Terminal,Leckie Laboratory,Judson Relay,West Hub"
+ },
+ {
+ "name": "Amuza",
+ "pos_x": 71.1875,
+ "pos_y": -88.1875,
+ "pos_z": 96.125,
+ "stations": "Bohme Dock,Dubyago Station,Uto Dock,Bauman Vision,Boss Orbital,Andoyer Vision,MacCurdy Beacon,Verne Settlement,Skjellerup Orbital,Jones Terminal,Monge Base,Alexeyev Bastion"
+ },
+ {
+ "name": "Amuzgo",
+ "pos_x": 146.40625,
+ "pos_y": -4.9375,
+ "pos_z": -16.28125,
+ "stations": "Geston Gateway,Samuda Settlement,King Enterprise,Regiomontanus Hub"
+ },
+ {
+ "name": "Amy-Charlotte",
+ "pos_x": -41.03125,
+ "pos_y": 41.96875,
+ "pos_z": -99.0625,
+ "stations": "Tezuka,Werber Camp"
+ },
+ {
+ "name": "Anagori",
+ "pos_x": 30.65625,
+ "pos_y": -14.90625,
+ "pos_z": 173.46875,
+ "stations": "Alcala Terminal,Gordon City,Ramsay City"
+ },
+ {
+ "name": "Anagorovici",
+ "pos_x": 13.3125,
+ "pos_y": -15.78125,
+ "pos_z": 170.03125,
+ "stations": "Wisniewski-Snerg Prospect,Coblentz Terminal"
+ },
+ {
+ "name": "Anagototo",
+ "pos_x": 58.125,
+ "pos_y": -18.8125,
+ "pos_z": -42.3125,
+ "stations": "Taylor Ring,Lee Hub,MacKellar Depot,Bean Installation,Laird Hub,Shepherd Enterprise"
+ },
+ {
+ "name": "Anahit",
+ "pos_x": 75.34375,
+ "pos_y": -138.9375,
+ "pos_z": 33.4375,
+ "stations": "Scorpio Midget,Shane Gleeson's Habitat,Penrose Arsenal,Turner's Inheritance,Payne-Gaposchkin Dock"
+ },
+ {
+ "name": "Anaia",
+ "pos_x": 90.375,
+ "pos_y": -213.78125,
+ "pos_z": -38.0625,
+ "stations": "Sato Orbital,Fraunhofer Mine,Rowley Beacon"
+ },
+ {
+ "name": "Anaiwan",
+ "pos_x": 97.0625,
+ "pos_y": -219.96875,
+ "pos_z": -31.40625,
+ "stations": "Lozino-Lozinskiy Stop,Mies van der Rohe Stop,Abnett Silo"
+ },
+ {
+ "name": "Anali",
+ "pos_x": -75.5625,
+ "pos_y": -15.9375,
+ "pos_z": 80,
+ "stations": "Lee Horizons,May City,Freycinet Enterprise"
+ },
+ {
+ "name": "Anana",
+ "pos_x": 9.65625,
+ "pos_y": -42,
+ "pos_z": 163.15625,
+ "stations": "Hooke Landing,Yamazaki Base"
+ },
+ {
+ "name": "Ananda",
+ "pos_x": 88.65625,
+ "pos_y": -99.53125,
+ "pos_z": 156.46875,
+ "stations": "Janjetov Port,Truman City,Elder Beacon,Fleming Depot,Meaney Hub"
+ },
+ {
+ "name": "Anandariti",
+ "pos_x": 153.6875,
+ "pos_y": 90.59375,
+ "pos_z": -45.03125,
+ "stations": "MacLean Mine"
+ },
+ {
+ "name": "Anandilla",
+ "pos_x": 100.40625,
+ "pos_y": 35.375,
+ "pos_z": 61.125,
+ "stations": "Volynov Landing,Grissom Point"
+ },
+ {
+ "name": "Anandini",
+ "pos_x": -129.25,
+ "pos_y": -24.8125,
+ "pos_z": 12.9375,
+ "stations": "Riemann Enterprise,Wallis Dock,Hennepin Terminal,Phillpotts Keep,Alpers Hub"
+ },
+ {
+ "name": "Anani",
+ "pos_x": 29.6875,
+ "pos_y": 105.09375,
+ "pos_z": -130.625,
+ "stations": "Adams Camp"
+ },
+ {
+ "name": "Ananke",
+ "pos_x": 4.40625,
+ "pos_y": 7.15625,
+ "pos_z": 57.5625,
+ "stations": "Cavendish Station,Shukor Observatory,Pawelczyk Arsenal"
+ },
+ {
+ "name": "Anantet",
+ "pos_x": 119.34375,
+ "pos_y": 13.1875,
+ "pos_z": 9.375,
+ "stations": "Cleave Port,Bischoff Camp,Buchli Plant"
+ },
+ {
+ "name": "Anapel",
+ "pos_x": 131,
+ "pos_y": 48.6875,
+ "pos_z": 34.34375,
+ "stations": "Oppenheimer Beacon,Hoffman's Folly"
+ },
+ {
+ "name": "Anapi",
+ "pos_x": -131.4375,
+ "pos_y": -13.84375,
+ "pos_z": 33.21875,
+ "stations": "Starzl Landing,Alexandria Settlement"
+ },
+ {
+ "name": "Anapos",
+ "pos_x": -53.5625,
+ "pos_y": 41.90625,
+ "pos_z": 41.75,
+ "stations": "Remek Platform,Herschel Plant"
+ },
+ {
+ "name": "Anapoya",
+ "pos_x": 56.75,
+ "pos_y": 75.25,
+ "pos_z": -64.75,
+ "stations": "Mendeleev Prospect,Kummer Dock,Hawkes Station"
+ },
+ {
+ "name": "Anareldt",
+ "pos_x": 56.5,
+ "pos_y": -20.75,
+ "pos_z": 116.9375,
+ "stations": "Wilhelm Camp"
+ },
+ {
+ "name": "Anari",
+ "pos_x": 81.875,
+ "pos_y": -56.5,
+ "pos_z": 105.65625,
+ "stations": "Bouvard City,Everest Colony,Brunner's Progress"
+ },
+ {
+ "name": "Anarina",
+ "pos_x": 101.75,
+ "pos_y": -64.125,
+ "pos_z": 89.71875,
+ "stations": "McKie Works,Moffat Landing,Cowling Vision,Naubakht Station"
+ },
+ {
+ "name": "Anarkusuga",
+ "pos_x": 124.125,
+ "pos_y": 90.4375,
+ "pos_z": -99.9375,
+ "stations": "Gloss Dock,Carrasco Orbital,Schiltberger Landing"
+ },
+ {
+ "name": "Anartoi",
+ "pos_x": 58.71875,
+ "pos_y": -172.3125,
+ "pos_z": 152.5625,
+ "stations": "Sheepshanks Colony,Brunner Prospect,Low Enterprise"
+ },
+ {
+ "name": "Anaruwa",
+ "pos_x": 55.90625,
+ "pos_y": 31.8125,
+ "pos_z": 88.34375,
+ "stations": "Fermi Prospect,Skvortsov Mines,Smith Barracks"
+ },
+ {
+ "name": "Anat",
+ "pos_x": 87.40625,
+ "pos_y": 79.125,
+ "pos_z": 110.0625,
+ "stations": "Ordway Beacon"
+ },
+ {
+ "name": "Anatahen",
+ "pos_x": 124.3125,
+ "pos_y": 78.09375,
+ "pos_z": -78.875,
+ "stations": "Madsen Settlement,Pinzon Relay"
+ },
+ {
+ "name": "Anates",
+ "pos_x": -69.84375,
+ "pos_y": 21.90625,
+ "pos_z": 132.5625,
+ "stations": "Bondar Terminal,Wellman Terminal"
+ },
+ {
+ "name": "Anayali",
+ "pos_x": 2.53125,
+ "pos_y": -67.8125,
+ "pos_z": 71.71875,
+ "stations": "Maughmer City,Tietjen Landing"
+ },
+ {
+ "name": "Anaye",
+ "pos_x": 123.9375,
+ "pos_y": -167.4375,
+ "pos_z": 48.65625,
+ "stations": "Roemer Hub,Ortiz Moreno Terminal,Gehry Beacon,Shunkai Enterprise,Bailey Silo"
+ },
+ {
+ "name": "Anayicates",
+ "pos_x": 113.03125,
+ "pos_y": -90.5,
+ "pos_z": 50.8125,
+ "stations": "Kushida Ring"
+ },
+ {
+ "name": "Anayol",
+ "pos_x": -30.84375,
+ "pos_y": 103.75,
+ "pos_z": -7.125,
+ "stations": "Andrey Popov Works,Wilkes Legacy,Ehrlich Legacy,Alexandrov Port"
+ },
+ {
+ "name": "Anca",
+ "pos_x": -14.71875,
+ "pos_y": -138,
+ "pos_z": -57.375,
+ "stations": "Schoenherr Orbital,Hogg Platform,Joy Vision,Kuttner Vision,Zetford Landing"
+ },
+ {
+ "name": "Ancalufon",
+ "pos_x": 106.875,
+ "pos_y": 43.09375,
+ "pos_z": 72,
+ "stations": "Feoktistov Orbital,Martinez Stop,Stasheff Bastion,Jolliet Beacon"
+ },
+ {
+ "name": "Ancanec",
+ "pos_x": 12.78125,
+ "pos_y": -58.5,
+ "pos_z": -97,
+ "stations": "Watts Horizons,Marshburn Bastion,Kepler Terminal"
+ },
+ {
+ "name": "Ancate",
+ "pos_x": 7.8125,
+ "pos_y": -155.6875,
+ "pos_z": 43.1875,
+ "stations": "Vogel Orbital,Challis Vision"
+ },
+ {
+ "name": "Ancento",
+ "pos_x": -104.875,
+ "pos_y": -73.96875,
+ "pos_z": 50.71875,
+ "stations": "O'Donnell Terminal,Longyear Horizons,Ellern Survey"
+ },
+ {
+ "name": "Ancermoten",
+ "pos_x": -73.9375,
+ "pos_y": -72.21875,
+ "pos_z": -129.75,
+ "stations": "Brandenstein Orbital,Davidson Base,von Helmont Installation,Onufriyenko City"
+ },
+ {
+ "name": "Ancha",
+ "pos_x": -99.90625,
+ "pos_y": -141.5625,
+ "pos_z": 71.59375,
+ "stations": "Frechet Port"
+ },
+ {
+ "name": "Andana",
+ "pos_x": -39.125,
+ "pos_y": 66.4375,
+ "pos_z": 100.0625,
+ "stations": "Dupuy de Lome's Exile"
+ },
+ {
+ "name": "Andancan",
+ "pos_x": -75,
+ "pos_y": 43,
+ "pos_z": -63,
+ "stations": "SVP"
+ },
+ {
+ "name": "Andarici",
+ "pos_x": 140.125,
+ "pos_y": -94.625,
+ "pos_z": 46.5,
+ "stations": "Esposito Terminal,Carlisle Horizons,Kubokawa Orbital,Lassell Port,Binney Vision"
+ },
+ {
+ "name": "Andavandul",
+ "pos_x": 90.6875,
+ "pos_y": 6.1875,
+ "pos_z": -83.09375,
+ "stations": "Searfoss Ring,Blenkinsop Hub"
+ },
+ {
+ "name": "Andceeth",
+ "pos_x": 70.78125,
+ "pos_y": -19.78125,
+ "pos_z": -8.28125,
+ "stations": "Hill's Folly,Wilde Refinery,Morris Station,Berners-Lee Legacy,Denver Station,Zetford Hub,Barcelos Reach,Vaez de Torres Terminal"
+ },
+ {
+ "name": "Ande",
+ "pos_x": 109.40625,
+ "pos_y": -140.4375,
+ "pos_z": -26.90625,
+ "stations": "Howe Vision,Lemaitre Station,Hay Vision,Qian's Progress,Shapley Works"
+ },
+ {
+ "name": "Andecavi",
+ "pos_x": 39.84375,
+ "pos_y": 42.15625,
+ "pos_z": -125.34375,
+ "stations": "Saavedra Platform"
+ },
+ {
+ "name": "Andel",
+ "pos_x": 75.21875,
+ "pos_y": 18.84375,
+ "pos_z": -48.34375,
+ "stations": "Sharp Dock,Serling Beacon,Baudin Arsenal"
+ },
+ {
+ "name": "Andenwi",
+ "pos_x": 140.84375,
+ "pos_y": -184.96875,
+ "pos_z": 17.875,
+ "stations": "d'Arrest Port,Kubokawa Camp,Reichelt Settlement"
+ },
+ {
+ "name": "Andere",
+ "pos_x": -63.21875,
+ "pos_y": 57.625,
+ "pos_z": -16,
+ "stations": "Friesner Port,Malzberg Vision,Kippax Terminal,Maury Terminal,McDevitt Vision,Kummer City,Low Works,Yang Settlement,Bethe Legacy"
+ },
+ {
+ "name": "Andhan",
+ "pos_x": 85.6875,
+ "pos_y": -96.65625,
+ "pos_z": 83.65625,
+ "stations": "Binney Dock,Yamamoto Port"
+ },
+ {
+ "name": "Andhantja",
+ "pos_x": -139.75,
+ "pos_y": -34.8125,
+ "pos_z": 123.8125,
+ "stations": "Crumey Orbital,Marley Horizons,VanderMeer Port,Heaviside Forum"
+ },
+ {
+ "name": "Andharas",
+ "pos_x": 149.40625,
+ "pos_y": 22.25,
+ "pos_z": -44.53125,
+ "stations": "Brunel Horizons,Paulmier de Gonneville Legacy"
+ },
+ {
+ "name": "Andharib",
+ "pos_x": 88.78125,
+ "pos_y": -104.96875,
+ "pos_z": 116.03125,
+ "stations": "Bentham Lab,Eddington Settlement,Young Port"
+ },
+ {
+ "name": "Andharveti",
+ "pos_x": -82.03125,
+ "pos_y": 19.34375,
+ "pos_z": 137.34375,
+ "stations": "Pierce Prospect"
+ },
+ {
+ "name": "Andhi",
+ "pos_x": 43.84375,
+ "pos_y": -93.5,
+ "pos_z": -55.15625,
+ "stations": "Ejeta Gateway,Meikle City,Hale Station,Santos Vision,Stabenow's Claim"
+ },
+ {
+ "name": "Andhrimi",
+ "pos_x": 10.375,
+ "pos_y": -54.40625,
+ "pos_z": 18.6875,
+ "stations": "Yurchikhin Station,Worlidge Enterprise,Fullerton Port,Ansari Terminal,Big Pappa's Base"
+ },
+ {
+ "name": "Andijin",
+ "pos_x": -46.8125,
+ "pos_y": 81,
+ "pos_z": 76.90625,
+ "stations": "Chapman Hub"
+ },
+ {
+ "name": "Andine",
+ "pos_x": 45,
+ "pos_y": 129.46875,
+ "pos_z": -51.96875,
+ "stations": "Scortia Dock,Desargues Plant"
+ },
+ {
+ "name": "Andje",
+ "pos_x": 25.34375,
+ "pos_y": -33.75,
+ "pos_z": -84.625,
+ "stations": "Laird Colony,Van Scyoc Installation"
+ },
+ {
+ "name": "Andjeri",
+ "pos_x": -100.09375,
+ "pos_y": 27.1875,
+ "pos_z": -54.4375,
+ "stations": "Bulgarin Dock,Temple Vision,Lopez de Villalobos Point"
+ },
+ {
+ "name": "Andlit",
+ "pos_x": -99.15625,
+ "pos_y": 14.1875,
+ "pos_z": 6.53125,
+ "stations": "Hilmers Ring,Coblentz Terminal,Copernicus Terminal,Henry Enterprise,O'Brien Barracks"
+ },
+ {
+ "name": "Andonnus",
+ "pos_x": 165.875,
+ "pos_y": -69.15625,
+ "pos_z": 54.46875,
+ "stations": "Mrkos Vision,Bessel Orbital,Cartan Point,Kube-McDowell Legacy,Dittmar Ring"
+ },
+ {
+ "name": "Andoque",
+ "pos_x": 136.5,
+ "pos_y": -166.5625,
+ "pos_z": -3.4375,
+ "stations": "Brorsen Dock"
+ },
+ {
+ "name": "Andowa",
+ "pos_x": 146.25,
+ "pos_y": 68.5625,
+ "pos_z": 18.40625,
+ "stations": "Martins Survey,Ahern Platform,Gardner Orbital"
+ },
+ {
+ "name": "Andowathaku",
+ "pos_x": 27.25,
+ "pos_y": -74.5,
+ "pos_z": -19.0625,
+ "stations": "Berliner Terminal,Stanier Settlement,Ciferri Settlement"
+ },
+ {
+ "name": "Andowatye",
+ "pos_x": 1.84375,
+ "pos_y": -10.09375,
+ "pos_z": 94.1875,
+ "stations": "Bosch Station,Born Terminal,Watson Hub,Cameron Ring,Steinmuller Observatory"
+ },
+ {
+ "name": "Andr",
+ "pos_x": 86.125,
+ "pos_y": -112.40625,
+ "pos_z": -43.09375,
+ "stations": "Giraud Colony,Finlay Terminal"
+ },
+ {
+ "name": "Andra",
+ "pos_x": 25,
+ "pos_y": 137.78125,
+ "pos_z": -43.375,
+ "stations": "Dantec Plant"
+ },
+ {
+ "name": "Andracanike",
+ "pos_x": 9.0625,
+ "pos_y": 62.875,
+ "pos_z": 159.75,
+ "stations": "Love Dock"
+ },
+ {
+ "name": "Andracottii",
+ "pos_x": -23.03125,
+ "pos_y": -136.9375,
+ "pos_z": -37.625,
+ "stations": "Bessel's Inheritance,Rich Platform,McMillan Settlement"
+ },
+ {
+ "name": "Andriani",
+ "pos_x": 161.90625,
+ "pos_y": -158.84375,
+ "pos_z": 114.59375,
+ "stations": "Lockyer Ring,Kanai Gateway,Suntzeff City,Payne Installation,Lunan Enterprise"
+ },
+ {
+ "name": "Andrungarri",
+ "pos_x": -70.75,
+ "pos_y": -10.03125,
+ "pos_z": 139.5,
+ "stations": "Altshuller Relay,Disch Port,Reis Ring,Maddox Dock"
+ },
+ {
+ "name": "Anduliga",
+ "pos_x": 124.625,
+ "pos_y": 2.5,
+ "pos_z": 61.25,
+ "stations": "Nolan Hub,Celsius Estate"
+ },
+ {
+ "name": "Andvaris",
+ "pos_x": -41.5625,
+ "pos_y": 42.1875,
+ "pos_z": 117,
+ "stations": "Wolfe Dock,de Andrade Park"
+ },
+ {
+ "name": "Andvarites",
+ "pos_x": 38.34375,
+ "pos_y": 7.875,
+ "pos_z": 86.65625,
+ "stations": "Piper Platform,Priestley Orbital,Kovalevsky Reach"
+ },
+ {
+ "name": "Andvaroges",
+ "pos_x": -80.28125,
+ "pos_y": -15.1875,
+ "pos_z": -80.03125,
+ "stations": "Deb Port,Pavlou Base,Flade Dock"
+ },
+ {
+ "name": "Ane",
+ "pos_x": 126.5625,
+ "pos_y": -168.34375,
+ "pos_z": -44.8125,
+ "stations": "Hogg Terminal"
+ },
+ {
+ "name": "Anedjet",
+ "pos_x": -102.625,
+ "pos_y": 98,
+ "pos_z": -2.84375,
+ "stations": "Barbosa Station,Herbert Enterprise,McQuay Station,Heck Installation,Tevis Works"
+ },
+ {
+ "name": "Anedjete",
+ "pos_x": -50.03125,
+ "pos_y": -105.5625,
+ "pos_z": 41.875,
+ "stations": "Struzan Hub,Bacigalupi Palace,Davies Ring,Schroeder Colony"
+ },
+ {
+ "name": "Anek Wang",
+ "pos_x": 77.34375,
+ "pos_y": 78.21875,
+ "pos_z": 49.71875,
+ "stations": "Henize Hub"
+ },
+ {
+ "name": "Anek Wango",
+ "pos_x": 93.71875,
+ "pos_y": -51.03125,
+ "pos_z": -3.0625,
+ "stations": "Szameit Landing,Gibson Survey,Roed Odegaard Landing,Lloyd Wright Base"
+ },
+ {
+ "name": "Anekaliti",
+ "pos_x": 18.25,
+ "pos_y": -39.09375,
+ "pos_z": 36.9375,
+ "stations": "Bean Terminal,Hutton Park,Potrykus Gateway"
+ },
+ {
+ "name": "Anekpenges",
+ "pos_x": -89.96875,
+ "pos_y": 17.9375,
+ "pos_z": -117.28125,
+ "stations": "Sharman Port,Vesalius Terminal"
+ },
+ {
+ "name": "Anemoi",
+ "pos_x": -61.375,
+ "pos_y": 22.25,
+ "pos_z": 1.5625,
+ "stations": "Gresley Port,Beadle Landing"
+ },
+ {
+ "name": "Aneti",
+ "pos_x": 127.875,
+ "pos_y": 56.25,
+ "pos_z": -39.625,
+ "stations": "Shuttleworth Enterprise,Linteris Dock"
+ },
+ {
+ "name": "Anetiman",
+ "pos_x": 156.625,
+ "pos_y": -155,
+ "pos_z": 51.65625,
+ "stations": "Brill Sanctuary,Pacheco Point"
+ },
+ {
+ "name": "Anets",
+ "pos_x": -3.15625,
+ "pos_y": -168.4375,
+ "pos_z": 49,
+ "stations": "Wegner Terminal,Kizawa Port"
+ },
+ {
+ "name": "Anezgan",
+ "pos_x": -88.59375,
+ "pos_y": 10.40625,
+ "pos_z": -71.28125,
+ "stations": "Linnehan Ring,Mendez Terminal,Apt City,Arber Orbital,Carter Orbital,Hertz Hub,Hoyle Settlement,Popov Dock,Menezes Survey,Carr Works"
+ },
+ {
+ "name": "Angakutasa",
+ "pos_x": -90.9375,
+ "pos_y": -87.8125,
+ "pos_z": -100.15625,
+ "stations": "Still Dock,Moseley Park,King Enterprise,Ivins Installation"
+ },
+ {
+ "name": "Angalkuq",
+ "pos_x": -121.8125,
+ "pos_y": 104.46875,
+ "pos_z": -64.375,
+ "stations": "Nasmyth Dock"
+ },
+ {
+ "name": "Angalufon",
+ "pos_x": -20.8125,
+ "pos_y": -177.9375,
+ "pos_z": 85.375,
+ "stations": "Berkey Relay,Wendelin Laboratory"
+ },
+ {
+ "name": "Angaluk",
+ "pos_x": 48.8125,
+ "pos_y": -95.0625,
+ "pos_z": -86.21875,
+ "stations": "Lynden-Bell Escape,Utley Outpost,H. G. Wells Port"
+ },
+ {
+ "name": "Anganaha",
+ "pos_x": 58,
+ "pos_y": -130.84375,
+ "pos_z": -27.3125,
+ "stations": "Pangborn Reach,Vogel Enterprise,Hague Settlement"
+ },
+ {
+ "name": "Angandracab",
+ "pos_x": 124.1875,
+ "pos_y": -59.9375,
+ "pos_z": 31.1875,
+ "stations": "Anderson Survey"
+ },
+ {
+ "name": "Angardh",
+ "pos_x": 178.90625,
+ "pos_y": -165.90625,
+ "pos_z": 64.375,
+ "stations": "Hiyya Ring,Freas Hub,Tedin Vision"
+ },
+ {
+ "name": "Angatic",
+ "pos_x": -64.4375,
+ "pos_y": -144.15625,
+ "pos_z": 40.03125,
+ "stations": "Springer Base"
+ },
+ {
+ "name": "Angawal",
+ "pos_x": -47,
+ "pos_y": -40.65625,
+ "pos_z": -85.5625,
+ "stations": "Popov Depot,Pinto Settlement,Serrao Beacon"
+ },
+ {
+ "name": "Angawu Lin",
+ "pos_x": -120.90625,
+ "pos_y": 71.09375,
+ "pos_z": 37.21875,
+ "stations": "Rodrigues Vision,Scortia Base"
+ },
+ {
+ "name": "Angen",
+ "pos_x": 84.6875,
+ "pos_y": 123.03125,
+ "pos_z": -47.21875,
+ "stations": "Smith Landing,Back Dock"
+ },
+ {
+ "name": "Anges",
+ "pos_x": 6.25,
+ "pos_y": -14.8125,
+ "pos_z": -141.5,
+ "stations": "Savorgnan de Brazza Settlement,Bokeili Dock"
+ },
+ {
+ "name": "Angetenar",
+ "pos_x": 40.09375,
+ "pos_y": -164.3125,
+ "pos_z": -79.59375,
+ "stations": "Daqing Mines,Klink Landing,Fukushima Dock"
+ },
+ {
+ "name": "Angi",
+ "pos_x": 62,
+ "pos_y": -68.96875,
+ "pos_z": 36.375,
+ "stations": "Georg Bothe Beacon,Lerner Base,Khan Port,Rondon's Progress"
+ },
+ {
+ "name": "Anginti",
+ "pos_x": 160.96875,
+ "pos_y": 36.4375,
+ "pos_z": -36.1875,
+ "stations": "Scobee Dock"
+ },
+ {
+ "name": "Angirasa",
+ "pos_x": 71.625,
+ "pos_y": 44.21875,
+ "pos_z": 140.125,
+ "stations": "Bergerac Mine"
+ },
+ {
+ "name": "Anglii",
+ "pos_x": 10.3125,
+ "pos_y": 36.3125,
+ "pos_z": -60.21875,
+ "stations": "Starzl Plant,Vizcaino Keep"
+ },
+ {
+ "name": "Angokomu",
+ "pos_x": 76.25,
+ "pos_y": -244.5,
+ "pos_z": 65.25,
+ "stations": "Mooz Vision,Ahnert Relay"
+ },
+ {
+ "name": "Angra",
+ "pos_x": 22.625,
+ "pos_y": -71.5625,
+ "pos_z": -64.90625,
+ "stations": "Barreiros Relay,Kennan Station"
+ },
+ {
+ "name": "Angrboldoga",
+ "pos_x": -118.5,
+ "pos_y": 87.53125,
+ "pos_z": 8.65625,
+ "stations": "Hovell Station,Cardano Orbital,Alvares Installation,Asher Relay"
+ },
+ {
+ "name": "Angrbole",
+ "pos_x": -9.90625,
+ "pos_y": -193.9375,
+ "pos_z": 93.5625,
+ "stations": "Leuschner's Claim"
+ },
+ {
+ "name": "Angrbon",
+ "pos_x": 89.9375,
+ "pos_y": -188.34375,
+ "pos_z": 115.96875,
+ "stations": "Kagawa Outpost"
+ },
+ {
+ "name": "Angrbonii",
+ "pos_x": 61.65625,
+ "pos_y": -42.4375,
+ "pos_z": 53.59375,
+ "stations": "Brash Refinery,Gooch City,Ewald Port,Kondakova Vision"
+ },
+ {
+ "name": "Angua",
+ "pos_x": 137.8125,
+ "pos_y": 48.71875,
+ "pos_z": 17.5625,
+ "stations": "Ray Depot"
+ },
+ {
+ "name": "Angui",
+ "pos_x": 28.34375,
+ "pos_y": 60.59375,
+ "pos_z": -149.4375,
+ "stations": "Rayhan al-Biruni Port,Houtman Base,Gunn Relay,Laird Hub"
+ },
+ {
+ "name": "Angurongo",
+ "pos_x": 72.6875,
+ "pos_y": -159.375,
+ "pos_z": 31.28125,
+ "stations": "Hopkinson Bastion,Jekhowsky Station,Dahm Port,Brooks Terminal"
+ },
+ {
+ "name": "Anhualm",
+ "pos_x": 94.5625,
+ "pos_y": -182.03125,
+ "pos_z": 67.5625,
+ "stations": "Deutsch Orbital,Knorre Beacon,Sunyaev Park,Check Vision,Nahavandi Vision"
+ },
+ {
+ "name": "Anhuanga",
+ "pos_x": 142.5,
+ "pos_y": -98.15625,
+ "pos_z": 3.65625,
+ "stations": "Hughes Installation,Arzachel City,Jones Platform"
+ },
+ {
+ "name": "Anhurs",
+ "pos_x": 114.8125,
+ "pos_y": -178.78125,
+ "pos_z": 73.0625,
+ "stations": "Axon Terminal,Chiang City,Riess Hub"
+ },
+ {
+ "name": "Anima",
+ "pos_x": 57.65625,
+ "pos_y": -61.1875,
+ "pos_z": -36.53125,
+ "stations": "Cowper Dock,Lind Silo,Baxter Enterprise"
+ },
+ {
+ "name": "Anindari",
+ "pos_x": 18.09375,
+ "pos_y": 42.53125,
+ "pos_z": 72,
+ "stations": "Stableford Orbital,Rangarajan Station"
+ },
+ {
+ "name": "Aning",
+ "pos_x": -39.65625,
+ "pos_y": -73.09375,
+ "pos_z": 51.3125,
+ "stations": "Banks Prospect"
+ },
+ {
+ "name": "Aningan",
+ "pos_x": -106.8125,
+ "pos_y": 16.25,
+ "pos_z": -107.21875,
+ "stations": "Wiberg Platform,Agassiz Terminal"
+ },
+ {
+ "name": "Aninohanu",
+ "pos_x": 17.125,
+ "pos_y": -75.125,
+ "pos_z": 7.09375,
+ "stations": "Avicenna Refinery,Bardeen Hangar"
+ },
+ {
+ "name": "Aninohini",
+ "pos_x": -119.625,
+ "pos_y": 10.375,
+ "pos_z": -32.375,
+ "stations": "Griffith Orbital,Sylvester Relay,Doi Beacon"
+ },
+ {
+ "name": "Anja",
+ "pos_x": 57.09375,
+ "pos_y": -176.75,
+ "pos_z": -61.9375,
+ "stations": "van Rhijn Station,Chalker's Inheritance,Alden Vision,Leberecht Tempel Vision,Caidin Legacy"
+ },
+ {
+ "name": "Anjana",
+ "pos_x": 27.84375,
+ "pos_y": 100.3125,
+ "pos_z": 121.15625,
+ "stations": "Saavedra Prospect,Powers Point,Ryman Depot"
+ },
+ {
+ "name": "Anka",
+ "pos_x": 41.78125,
+ "pos_y": -4.71875,
+ "pos_z": -103.78125,
+ "stations": "Malerba Landing,Howe Refinery,Volta Refinery"
+ },
+ {
+ "name": "Ankarawai",
+ "pos_x": 91.875,
+ "pos_y": -241.71875,
+ "pos_z": 63.125,
+ "stations": "van den Bergh Mine"
+ },
+ {
+ "name": "Ankata",
+ "pos_x": 156.09375,
+ "pos_y": -3.1875,
+ "pos_z": 25.25,
+ "stations": "Obruchev Vision,Skolem Orbital,Card Landing"
+ },
+ {
+ "name": "Ankayo",
+ "pos_x": 78.5,
+ "pos_y": -207.40625,
+ "pos_z": 80.96875,
+ "stations": "Derekas Outpost"
+ },
+ {
+ "name": "Ankou",
+ "pos_x": 95.1875,
+ "pos_y": -90.5,
+ "pos_z": 14.65625,
+ "stations": "Bao Terminal,Kirby Orbital,Anthony Point"
+ },
+ {
+ "name": "Anlave",
+ "pos_x": -25.375,
+ "pos_y": 1.125,
+ "pos_z": -20.84375,
+ "stations": "Brunton Gateway,Suri Park,Kobayashi City,Hogg City,Vaugh's Inheritance,Bain Dock,Roosa Forum,Viete Landing"
+ },
+ {
+ "name": "Anmaia",
+ "pos_x": 83.15625,
+ "pos_y": -96.96875,
+ "pos_z": 139.5,
+ "stations": "Shea Station,Arzachel Station,van de Hulst Reach,Biggle Point"
+ },
+ {
+ "name": "Anmatyerre",
+ "pos_x": 64.5625,
+ "pos_y": -20.75,
+ "pos_z": -13.53125,
+ "stations": "Roberts Observatory,Bennett Beacon,Watson Port"
+ },
+ {
+ "name": "Anna Perenna",
+ "pos_x": 29.53125,
+ "pos_y": 29.34375,
+ "pos_z": -42,
+ "stations": "Watson Laboratory,Wisoff Holdings"
+ },
+ {
+ "name": "Annar",
+ "pos_x": 19.96875,
+ "pos_y": -132.9375,
+ "pos_z": 53.5625,
+ "stations": "Kirby Platform"
+ },
+ {
+ "name": "Annarr",
+ "pos_x": -13.625,
+ "pos_y": -78.625,
+ "pos_z": 50.53125,
+ "stations": "Rocklynne Settlement,Siodmak's Inheritance,Bachman Landing"
+ },
+ {
+ "name": "Annarthia",
+ "pos_x": 41.5,
+ "pos_y": -252.25,
+ "pos_z": 37.625,
+ "stations": "Kimura Hub,van den Hove Holdings,Przhevalsky Depot"
+ },
+ {
+ "name": "Annwn",
+ "pos_x": 75.0625,
+ "pos_y": -135.46875,
+ "pos_z": 19.84375,
+ "stations": "Gallimaufry,Baade Enterprise"
+ },
+ {
+ "name": "Anociti",
+ "pos_x": 26.96875,
+ "pos_y": -39.03125,
+ "pos_z": 116.5,
+ "stations": "Lerner Orbital,Harding Base"
+ },
+ {
+ "name": "Anotand",
+ "pos_x": -154.84375,
+ "pos_y": 10.3125,
+ "pos_z": 47.8125,
+ "stations": "Sullivan Orbital,Morgan Relay"
+ },
+ {
+ "name": "Anotchadiae",
+ "pos_x": 72.4375,
+ "pos_y": 69.5625,
+ "pos_z": 58.40625,
+ "stations": "Malerba Orbital,Pawelczyk Hub,Crown Dock,Bujold Terminal"
+ },
+ {
+ "name": "Anotche",
+ "pos_x": 88.59375,
+ "pos_y": 76.59375,
+ "pos_z": -53.34375,
+ "stations": "Deb Base,Binnie Settlement,Fourneyron Orbital"
+ },
+ {
+ "name": "Anotchi",
+ "pos_x": 143.5625,
+ "pos_y": -203.40625,
+ "pos_z": 120.59375,
+ "stations": "Mukai Landing,Cuffey Holdings,Skolem Installation,Schwarzschild Beacon"
+ },
+ {
+ "name": "Anototo",
+ "pos_x": 172.75,
+ "pos_y": -21.03125,
+ "pos_z": -58.90625,
+ "stations": "McKee Works"
+ },
+ {
+ "name": "Anotribe",
+ "pos_x": 25.4375,
+ "pos_y": -159.0625,
+ "pos_z": -81.875,
+ "stations": "Kuchemann Port,Jones Port,Grzimek's Progress"
+ },
+ {
+ "name": "Anouke",
+ "pos_x": 173.25,
+ "pos_y": -77.59375,
+ "pos_z": 86.125,
+ "stations": "Lundmark Holdings,Pu Orbital,Garrett Refinery"
+ },
+ {
+ "name": "Anoukh",
+ "pos_x": 176.84375,
+ "pos_y": -138.96875,
+ "pos_z": 45.15625,
+ "stations": "Bainbridge's Progress"
+ },
+ {
+ "name": "Anoukha",
+ "pos_x": -22.53125,
+ "pos_y": 80.78125,
+ "pos_z": 149.53125,
+ "stations": "Ahmed Terminal,Young Orbital"
+ },
+ {
+ "name": "Anoumis",
+ "pos_x": -124.21875,
+ "pos_y": 102.90625,
+ "pos_z": 16.46875,
+ "stations": "Dowling Port,Mohun Arena"
+ },
+ {
+ "name": "Anouphis",
+ "pos_x": -97.53125,
+ "pos_y": 1.5,
+ "pos_z": 46.875,
+ "stations": "Anning Orbital,Kepler Terminal,Brongniart Terminal,Garratt Enterprise,Gemar Dock,Cayley Terminal,Dyomin Terminal,Watts Depot,MacDonald Survey,So-yeon Enterprise,Nowak Orbital,Foucault Port"
+ },
+ {
+ "name": "Ansari",
+ "pos_x": -84.5,
+ "pos_y": 37.59375,
+ "pos_z": -44.71875,
+ "stations": "Crown Installation,Cormack Horizons"
+ },
+ {
+ "name": "Ansuz",
+ "pos_x": 61.90625,
+ "pos_y": 5.96875,
+ "pos_z": 54.53125,
+ "stations": "Nelder Mine,Marconi Hangar,Berners-Lee Platform,Gantt Colony"
+ },
+ {
+ "name": "Antai",
+ "pos_x": 94.9375,
+ "pos_y": -44.65625,
+ "pos_z": -16.09375,
+ "stations": "Edwards Stop"
+ },
+ {
+ "name": "Antal",
+ "pos_x": -142.5,
+ "pos_y": -91.375,
+ "pos_z": -66.03125,
+ "stations": "Lobachevsky Station,Wagner Gateway,Charnas Dock"
+ },
+ {
+ "name": "Antan",
+ "pos_x": 120.46875,
+ "pos_y": -155.96875,
+ "pos_z": 37.5,
+ "stations": "Tago Outpost"
+ },
+ {
+ "name": "Antanasata",
+ "pos_x": -183.84375,
+ "pos_y": -55.21875,
+ "pos_z": 38.78125,
+ "stations": "Forsskal Dock"
+ },
+ {
+ "name": "Antani",
+ "pos_x": 89.625,
+ "pos_y": -205.84375,
+ "pos_z": 50.90625,
+ "stations": "Luyten's Exile"
+ },
+ {
+ "name": "Antanoai",
+ "pos_x": 29.78125,
+ "pos_y": -241.90625,
+ "pos_z": 56.1875,
+ "stations": "Gasparis Ring"
+ },
+ {
+ "name": "Antanyamai",
+ "pos_x": -103.875,
+ "pos_y": 0.1875,
+ "pos_z": 83.1875,
+ "stations": "Macomb City,Robinson Gateway,Cook Terminal"
+ },
+ {
+ "name": "Anteradabal",
+ "pos_x": 58.65625,
+ "pos_y": 119.8125,
+ "pos_z": -118.09375,
+ "stations": "Lie Barracks,Watts Enterprise,Favier Ring"
+ },
+ {
+ "name": "Antevorta",
+ "pos_x": 43.1875,
+ "pos_y": 39.3125,
+ "pos_z": 1.53125,
+ "stations": "Lebedev Ring,Vonarburg's Inheritance,Rorschach Gateway"
+ },
+ {
+ "name": "Antiang",
+ "pos_x": 107.59375,
+ "pos_y": -205.25,
+ "pos_z": 24.6875,
+ "stations": "Kresak Camp,Payne Lab"
+ },
+ {
+ "name": "Anticulosk",
+ "pos_x": -22.15625,
+ "pos_y": -63.40625,
+ "pos_z": 95.8125,
+ "stations": "Delbruck Ring,Penn's Progress"
+ },
+ {
+ "name": "Antikiriya",
+ "pos_x": 168.625,
+ "pos_y": -159.75,
+ "pos_z": 36.625,
+ "stations": "Young Terminal,Carroll Penal colony"
+ },
+ {
+ "name": "Antilope",
+ "pos_x": -89.53125,
+ "pos_y": -27.625,
+ "pos_z": 20.8125,
+ "stations": "Laing Dock,Irens Terminal,Shaikh City,Lee Silo,Dall Port,Betancourt Station,Tavares Ring,Thagard Landing,Midgeley Prospect,Slusser's Progress"
+ },
+ {
+ "name": "Antinica",
+ "pos_x": 64.5,
+ "pos_y": 62.3125,
+ "pos_z": -12.625,
+ "stations": "Akers Vision,Williamson Prospect"
+ },
+ {
+ "name": "Antliae Sector BQ-Y c12",
+ "pos_x": 146.46875,
+ "pos_y": 87.1875,
+ "pos_z": 8.75,
+ "stations": "Ogden Refinery"
+ },
+ {
+ "name": "Antliae Sector BQ-Y c22",
+ "pos_x": 156.71875,
+ "pos_y": 87.3125,
+ "pos_z": 5.125,
+ "stations": "Levchenko Enterprise"
+ },
+ {
+ "name": "Antliae Sector CL-Y d76",
+ "pos_x": 139.875,
+ "pos_y": 115.25,
+ "pos_z": 44.6875,
+ "stations": "West Arena,Brooks Observatory"
+ },
+ {
+ "name": "Antliae Sector CL-Y d82",
+ "pos_x": 172.21875,
+ "pos_y": 57.0625,
+ "pos_z": -7.8125,
+ "stations": "Nielsen's Progress"
+ },
+ {
+ "name": "Antliae Sector CL-Y d89",
+ "pos_x": 138.3125,
+ "pos_y": 62.21875,
+ "pos_z": 53.3125,
+ "stations": "Lorrah's Inheritance,Reynolds Hub,Farmer Landing"
+ },
+ {
+ "name": "Antliae Sector CL-Y d90",
+ "pos_x": 143.21875,
+ "pos_y": 105.625,
+ "pos_z": -12.75,
+ "stations": "Hiroyuki Terminal"
+ },
+ {
+ "name": "Antliae Sector CQ-Y b3",
+ "pos_x": 135,
+ "pos_y": 44.90625,
+ "pos_z": -7,
+ "stations": "Webb's Inheritance"
+ },
+ {
+ "name": "Antliae Sector DL-Y c4",
+ "pos_x": 135.25,
+ "pos_y": 36.78125,
+ "pos_z": -0.5625,
+ "stations": "Cixin Penal colony,Narbeth Base"
+ },
+ {
+ "name": "Antliae Sector EG-Y d134",
+ "pos_x": 152.5,
+ "pos_y": 11.78125,
+ "pos_z": 36.5,
+ "stations": "Tepper Refinery,Remek Silo"
+ },
+ {
+ "name": "Antliae Sector EG-Y d78",
+ "pos_x": 162.46875,
+ "pos_y": 33.15625,
+ "pos_z": 36.46875,
+ "stations": "Laplace Relay"
+ },
+ {
+ "name": "Antliae Sector EG-Y d80",
+ "pos_x": 173.75,
+ "pos_y": 23.09375,
+ "pos_z": 7.4375,
+ "stations": "Blodgett Holdings"
+ },
+ {
+ "name": "Antliae Sector FB-X b1-1",
+ "pos_x": 159.28125,
+ "pos_y": 58.1875,
+ "pos_z": 2.375,
+ "stations": "Qian Base,Makarov Depot"
+ },
+ {
+ "name": "Antliae Sector FL-Y b4",
+ "pos_x": 174.5625,
+ "pos_y": 33.9375,
+ "pos_z": -23.53125,
+ "stations": "Chern Vision"
+ },
+ {
+ "name": "Antliae Sector FL-Y b6",
+ "pos_x": 168,
+ "pos_y": 19.53125,
+ "pos_z": -22.21875,
+ "stations": "Lobachevsky's Folly"
+ },
+ {
+ "name": "Antliae Sector FW-W c1-19",
+ "pos_x": 170.21875,
+ "pos_y": 92.09375,
+ "pos_z": 27.75,
+ "stations": "Bertin Settlement"
+ },
+ {
+ "name": "Antliae Sector GR-W d1-83",
+ "pos_x": 156.65625,
+ "pos_y": 86.28125,
+ "pos_z": 87.75,
+ "stations": "Priest's Folly"
+ },
+ {
+ "name": "Antliae Sector HR-W c1-14",
+ "pos_x": 161.40625,
+ "pos_y": 45.34375,
+ "pos_z": 44.90625,
+ "stations": "Phillips Penal colony,Fulton Laboratory"
+ },
+ {
+ "name": "Antliae Sector HR-W c1-21",
+ "pos_x": 165.0625,
+ "pos_y": 48.65625,
+ "pos_z": 15.25,
+ "stations": "Makeev Refinery"
+ },
+ {
+ "name": "Antliae Sector HR-W c1-25",
+ "pos_x": 155.8125,
+ "pos_y": 43.96875,
+ "pos_z": 52.1875,
+ "stations": "Garcia Prospect"
+ },
+ {
+ "name": "Antliae Sector HR-W c1-3",
+ "pos_x": 153.625,
+ "pos_y": 24.5,
+ "pos_z": 24.90625,
+ "stations": "Pirsan's Inheritance,Klimuk Base"
+ },
+ {
+ "name": "Antliae Sector HR-W c1-9",
+ "pos_x": 135.53125,
+ "pos_y": 51.71875,
+ "pos_z": 35.75,
+ "stations": "Tolstoi Survey"
+ },
+ {
+ "name": "Antliae Sector IM-W d1-103",
+ "pos_x": 157.0625,
+ "pos_y": 21.03125,
+ "pos_z": 68.0625,
+ "stations": "Edwards Keep,Carstensz Base"
+ },
+ {
+ "name": "Antliae Sector IM-W d1-104",
+ "pos_x": 159.71875,
+ "pos_y": 10.71875,
+ "pos_z": 64.375,
+ "stations": "Dall Penal colony,Morris Vision,Wheelock Base"
+ },
+ {
+ "name": "Antliae Sector IM-W d1-124",
+ "pos_x": 165.3125,
+ "pos_y": 31,
+ "pos_z": 62.8125,
+ "stations": "Fancher Vista"
+ },
+ {
+ "name": "Antliae Sector IM-W d1-127",
+ "pos_x": 154.21875,
+ "pos_y": 23.5,
+ "pos_z": 72.28125,
+ "stations": "Wul Enterprise"
+ },
+ {
+ "name": "Antliae Sector IM-W d1-129",
+ "pos_x": 150.71875,
+ "pos_y": 13.375,
+ "pos_z": 61.34375,
+ "stations": "Hausdorff Plant,Meredith Camp"
+ },
+ {
+ "name": "Antliae Sector IM-W d1-96",
+ "pos_x": 153.15625,
+ "pos_y": 47.4375,
+ "pos_z": 74.46875,
+ "stations": "Pryor Base"
+ },
+ {
+ "name": "Antliae Sector IM-W d1-97",
+ "pos_x": 151.125,
+ "pos_y": 53.40625,
+ "pos_z": 79.9375,
+ "stations": "Butler Holdings,Linge Works"
+ },
+ {
+ "name": "Antliae Sector JC-V c2-12",
+ "pos_x": 148.40625,
+ "pos_y": 55.46875,
+ "pos_z": 74.25,
+ "stations": "Oltion Base,Wagner's Folly,Gernsback Terminal"
+ },
+ {
+ "name": "Antliae Sector JC-V c2-19",
+ "pos_x": 155.5625,
+ "pos_y": 63.65625,
+ "pos_z": 61.9375,
+ "stations": "Lovell's Claim"
+ },
+ {
+ "name": "Antliae Sector JM-W c1-31",
+ "pos_x": 141.28125,
+ "pos_y": 13.09375,
+ "pos_z": 16.875,
+ "stations": "Keyes Penal colony,Thomson Plant"
+ },
+ {
+ "name": "Antliae Sector LX-U c2-8",
+ "pos_x": 151.84375,
+ "pos_y": 22.71875,
+ "pos_z": 59.40625,
+ "stations": "Due Base,Alvarado Installation"
+ },
+ {
+ "name": "Antliae Sector NX-U b2-4",
+ "pos_x": 155.90625,
+ "pos_y": 22.84375,
+ "pos_z": 23.28125,
+ "stations": "Alvares Installation"
+ },
+ {
+ "name": "Antliae Sector PI-T b3-7",
+ "pos_x": 171.5625,
+ "pos_y": 53.6875,
+ "pos_z": 54.4375,
+ "stations": "Macdonald Settlement"
+ },
+ {
+ "name": "Antliae Sector RD-T b3-3",
+ "pos_x": 158.71875,
+ "pos_y": 20.375,
+ "pos_z": 35.0625,
+ "stations": "Bujold Relay,Smith Terminal"
+ },
+ {
+ "name": "Antliae Sector SO-R b4-2",
+ "pos_x": 136.40625,
+ "pos_y": 47.0625,
+ "pos_z": 65.1875,
+ "stations": "Roberts Mines,Collins Point"
+ },
+ {
+ "name": "Antliae Sector SO-R b4-6",
+ "pos_x": 149.03125,
+ "pos_y": 43.28125,
+ "pos_z": 55.5,
+ "stations": "Heaviside Keep"
+ },
+ {
+ "name": "Antliae Sector TY-S b3-2",
+ "pos_x": 161.90625,
+ "pos_y": 7.5,
+ "pos_z": 39.15625,
+ "stations": "Bamford Enterprise"
+ },
+ {
+ "name": "Antliae Sector XJ-A c15",
+ "pos_x": 149.4375,
+ "pos_y": 82.6875,
+ "pos_z": -27.53125,
+ "stations": "Martins Prospect"
+ },
+ {
+ "name": "Antliae Sector ZE-A c25",
+ "pos_x": 169.09375,
+ "pos_y": 42.0625,
+ "pos_z": -31.875,
+ "stations": "Robinson Settlement"
+ },
+ {
+ "name": "Antobri",
+ "pos_x": -57.59375,
+ "pos_y": 23.46875,
+ "pos_z": -74.75,
+ "stations": "Treshchov Dock,Aleksandrov Terminal,Reynolds Beacon,Weston Dock,Wilmore Terminal,Conway Enterprise,Gidzenko City,Wolf Ring,Hardwick Depot"
+ },
+ {
+ "name": "Antove Den",
+ "pos_x": 55.1875,
+ "pos_y": -73.625,
+ "pos_z": -48.34375,
+ "stations": "Tall Dock,Dufay Lab"
+ },
+ {
+ "name": "Antovii",
+ "pos_x": -41.03125,
+ "pos_y": -132.03125,
+ "pos_z": 39.59375,
+ "stations": "Hevelius Landing,Ferguson Beacon,Rabinowitz Platform"
+ },
+ {
+ "name": "Anu",
+ "pos_x": -64.65625,
+ "pos_y": -19.09375,
+ "pos_z": -19.625,
+ "stations": "Lichtenberg Terminal,Irvin Plant,Schmitt Port"
+ },
+ {
+ "name": "Anukam",
+ "pos_x": 82.125,
+ "pos_y": -4.84375,
+ "pos_z": 1.1875,
+ "stations": "Stephenson Landing,Siodmak Holdings,Goddard Port,Bell Settlement"
+ },
+ {
+ "name": "Anukan",
+ "pos_x": 104.65625,
+ "pos_y": -52.78125,
+ "pos_z": -23.375,
+ "stations": "Florine Vision"
+ },
+ {
+ "name": "Anukas",
+ "pos_x": 148.4375,
+ "pos_y": 50.15625,
+ "pos_z": -7.375,
+ "stations": "Abbott City,Kennedy Enterprise,Cayley Vision,Narvaez Vision,Jolliet Beacon"
+ },
+ {
+ "name": "Anukh",
+ "pos_x": -17.25,
+ "pos_y": 139.90625,
+ "pos_z": -4.96875,
+ "stations": "Hovgaard Outpost,Maury Survey,Naddoddur Relay"
+ },
+ {
+ "name": "Anukul",
+ "pos_x": 4.5,
+ "pos_y": 100.4375,
+ "pos_z": 84.25,
+ "stations": "Somerset Depot,Szebehely Vision,Bulychev Beacon"
+ },
+ {
+ "name": "Anum",
+ "pos_x": 143,
+ "pos_y": -90.9375,
+ "pos_z": -18.8125,
+ "stations": "Dubyago Port,Cartier Gateway,Pierce Depot,Kraft Orbital"
+ },
+ {
+ "name": "Anuma",
+ "pos_x": 37.65625,
+ "pos_y": -59.71875,
+ "pos_z": 118.71875,
+ "stations": "Titov Depot"
+ },
+ {
+ "name": "Anumalan",
+ "pos_x": -75.8125,
+ "pos_y": -68.59375,
+ "pos_z": -10.46875,
+ "stations": "McMahon Orbital,Reis Port,Goonan's Folly,Carver Gateway"
+ },
+ {
+ "name": "Anumanas",
+ "pos_x": 150.59375,
+ "pos_y": -223.09375,
+ "pos_z": 56.9375,
+ "stations": "Hagihara Outpost,Oramus Camp"
+ },
+ {
+ "name": "Anumclaw",
+ "pos_x": 89,
+ "pos_y": -39.5625,
+ "pos_z": -72.21875,
+ "stations": "Jensen Ring,Kubasov City,Dupuy de Lome Silo,Zudov Survey"
+ },
+ {
+ "name": "Anurinjung",
+ "pos_x": -3.875,
+ "pos_y": -132.3125,
+ "pos_z": 60.09375,
+ "stations": "Kepler Vision,Flammarion Station,Roche Gateway,Hyecho Relay,Goldschmidt Hub,Tiedemann Relay,Carrington Horizons"
+ },
+ {
+ "name": "Anuron",
+ "pos_x": -42.1875,
+ "pos_y": -127.21875,
+ "pos_z": 124.65625,
+ "stations": "Lichtenberg Dock,Bond Dock,Cauchy's Inheritance"
+ },
+ {
+ "name": "Any Kutjara",
+ "pos_x": 13.46875,
+ "pos_y": -7.1875,
+ "pos_z": 143.0625,
+ "stations": "Metz Vision,Hugh Hub,Cantor Hub,Lindsey Palace,Williamson Gateway"
+ },
+ {
+ "name": "Any Minurok",
+ "pos_x": -109.78125,
+ "pos_y": -12.0625,
+ "pos_z": -124.28125,
+ "stations": "Overmyer Depot"
+ },
+ {
+ "name": "Any Na",
+ "pos_x": 125.65625,
+ "pos_y": -1.71875,
+ "pos_z": 14.09375,
+ "stations": "Libby Orbital,Pettit Port,McNair Ring"
+ },
+ {
+ "name": "Any Nal",
+ "pos_x": 85.34375,
+ "pos_y": -232.875,
+ "pos_z": -47.4375,
+ "stations": "Urkovic Colony,Hafner Station,Russo Beacon"
+ },
+ {
+ "name": "Anyama",
+ "pos_x": 87.125,
+ "pos_y": -154.3125,
+ "pos_z": -77.78125,
+ "stations": "i Sola's Inheritance,Inoda Sanctuary"
+ },
+ {
+ "name": "Anyanwu",
+ "pos_x": 32.15625,
+ "pos_y": 23.09375,
+ "pos_z": 32.1875,
+ "stations": "Bunsen Base,Delbruck Outpost"
+ },
+ {
+ "name": "Anyayahui",
+ "pos_x": 156.9375,
+ "pos_y": -15.84375,
+ "pos_z": 30.28125,
+ "stations": "Esposito Station,Antonov Station"
+ },
+ {
+ "name": "Anyayar",
+ "pos_x": 64.84375,
+ "pos_y": -158.8125,
+ "pos_z": -72.4375,
+ "stations": "Smith Survey,Reinmuth Landing,Francis Vision,Coulomb Base"
+ },
+ {
+ "name": "Ao",
+ "pos_x": 19.75,
+ "pos_y": 28.125,
+ "pos_z": 43.59375,
+ "stations": "Avicenna Hub,Parmitano Dock,Carstensz Laboratory,Eyharts Silo,Fleming City"
+ },
+ {
+ "name": "Ao Ch'an",
+ "pos_x": 86.21875,
+ "pos_y": -124.4375,
+ "pos_z": 106.125,
+ "stations": "al-Kashi Hub,Sargent Vision,Amis Enterprise,Cherry Station,Ayton Innes Dock,Rich Hub,Giacobini Hub,Saha Port,Helffrich Dock,Gorgani Dock,Farghani Colony,du Fresne Depot"
+ },
+ {
+ "name": "Ao Guang",
+ "pos_x": 0.625,
+ "pos_y": 40.03125,
+ "pos_z": -21.34375,
+ "stations": "H. G. Wells Plant,Sakers' Folly,Humphreys Barracks"
+ },
+ {
+ "name": "Ao Jung Mu",
+ "pos_x": 124.0625,
+ "pos_y": -63.625,
+ "pos_z": -47.71875,
+ "stations": "Borel Prospect,Bethke Installation,Barr Settlement,Broderick Settlement"
+ },
+ {
+ "name": "Ao Kach",
+ "pos_x": -112.5,
+ "pos_y": 69.71875,
+ "pos_z": -109.46875,
+ "stations": "Tall Outpost"
+ },
+ {
+ "name": "Ao Kang",
+ "pos_x": 32.375,
+ "pos_y": 18.28125,
+ "pos_z": -68.78125,
+ "stations": "Kinsey Ring,Mieville's Folly,Wells Relay,Zalyotin Penal colony"
+ },
+ {
+ "name": "Ao Kax",
+ "pos_x": 141.46875,
+ "pos_y": -21,
+ "pos_z": -64.0625,
+ "stations": "Babbage Horizons,Fermat Forum,Aristotle Orbital,Sellers Terminal"
+ },
+ {
+ "name": "Ao Kiang",
+ "pos_x": 147.65625,
+ "pos_y": 23.875,
+ "pos_z": -74.40625,
+ "stations": "Meade Landing,Cavalieri Stop"
+ },
+ {
+ "name": "Ao Kicho",
+ "pos_x": 0.34375,
+ "pos_y": -199.9375,
+ "pos_z": -40.25,
+ "stations": "Somayaji Dock,Hansen Mines,Wendelin's Inheritance"
+ },
+ {
+ "name": "Ao Konbul",
+ "pos_x": 68.65625,
+ "pos_y": 93,
+ "pos_z": 44.75,
+ "stations": "Peirce Freeport"
+ },
+ {
+ "name": "Ao Kond",
+ "pos_x": 60.96875,
+ "pos_y": 3.9375,
+ "pos_z": 116.40625,
+ "stations": "Fettman Enterprise"
+ },
+ {
+ "name": "Ao Kou",
+ "pos_x": 111.71875,
+ "pos_y": -171.5,
+ "pos_z": -51.71875,
+ "stations": "Pennington Platform,Auwers Point"
+ },
+ {
+ "name": "Ao Kuang",
+ "pos_x": -20.25,
+ "pos_y": 52.25,
+ "pos_z": -117.25,
+ "stations": "Plante Mines,Ponce de Leon Mines"
+ },
+ {
+ "name": "Ao Qin",
+ "pos_x": -62.90625,
+ "pos_y": 46.78125,
+ "pos_z": 1.53125,
+ "stations": "Brunner Horizons,Wandrei Silo,Smith Laboratory,Bohnhoff Orbital,Poisson Camp"
+ },
+ {
+ "name": "Ao Run",
+ "pos_x": -52.625,
+ "pos_y": -56.28125,
+ "pos_z": 15.78125,
+ "stations": "Pailes Landing,Eratosthenes Platform"
+ },
+ {
+ "name": "Ao Shun",
+ "pos_x": -75.3125,
+ "pos_y": 22.59375,
+ "pos_z": 1.09375,
+ "stations": "Pawelczyk Terminal,Rzeppa Gateway,Chu Depot,Filipchenko Prospect"
+ },
+ {
+ "name": "Aobrigen Nu",
+ "pos_x": 140.03125,
+ "pos_y": -5.25,
+ "pos_z": 52.21875,
+ "stations": "Hussenot Landing,Fleming Platform"
+ },
+ {
+ "name": "Aobriges",
+ "pos_x": 138.625,
+ "pos_y": -60.5625,
+ "pos_z": 36.21875,
+ "stations": "Stiles Platform,Burnham Dock,Whitelaw Base,Hunt Keep"
+ },
+ {
+ "name": "Aobriguana",
+ "pos_x": 103.0625,
+ "pos_y": -1.40625,
+ "pos_z": -97.40625,
+ "stations": "Ciferri Dock,Bacon Mines,Kovalyonok Oasis"
+ },
+ {
+ "name": "Aobriguites",
+ "pos_x": -139.90625,
+ "pos_y": 114.625,
+ "pos_z": 18.40625,
+ "stations": "Watt-Evans Relay"
+ },
+ {
+ "name": "Aobrogii",
+ "pos_x": -67.6875,
+ "pos_y": 18.8125,
+ "pos_z": 89.34375,
+ "stations": "Seddon Terminal,Williams Hub,Somerset Hub,Thuot Hub,Alvares' Progress"
+ },
+ {
+ "name": "Aong",
+ "pos_x": 65.0625,
+ "pos_y": 56.625,
+ "pos_z": 131.03125,
+ "stations": "Isherwood Landing,Webb's Inheritance,Williams Depot"
+ },
+ {
+ "name": "Aonga",
+ "pos_x": -34.46875,
+ "pos_y": 9.53125,
+ "pos_z": -90.875,
+ "stations": "Scott Terminal,Jernigan Terminal,Arnold Station,Carr Depot,Gooch Observatory,Boulle Prospect"
+ },
+ {
+ "name": "Aonghus",
+ "pos_x": -5.65625,
+ "pos_y": -161.625,
+ "pos_z": 105.71875,
+ "stations": "Palisa Mines,Phillips Survey"
+ },
+ {
+ "name": "Aongi",
+ "pos_x": 5.125,
+ "pos_y": -32.4375,
+ "pos_z": -64.8125,
+ "stations": "Nelson City"
+ },
+ {
+ "name": "Aonibozo",
+ "pos_x": -124.4375,
+ "pos_y": -60.75,
+ "pos_z": 70.9375,
+ "stations": "Reed Mine,Deb Base"
+ },
+ {
+ "name": "Aonii",
+ "pos_x": -2.34375,
+ "pos_y": -167.03125,
+ "pos_z": 11.75,
+ "stations": "Keeler City,Jackson Port,Mainzer City,Aoki Ring,Hickman Terminal,Lowell Dock,Offutt Penal colony,Westerhout Terminal,Arnold Prospect,Quimper Base,Menezes Installation,Giraud Holdings"
+ },
+ {
+ "name": "Aoniken",
+ "pos_x": 128.625,
+ "pos_y": 85.65625,
+ "pos_z": -58.15625,
+ "stations": "Dann Mine,Makarov Point"
+ },
+ {
+ "name": "Aonikenk",
+ "pos_x": -88.96875,
+ "pos_y": -79.5625,
+ "pos_z": -62.34375,
+ "stations": "Martins Enterprise,Qian Market,Compton Dock,Hasse Hub,Ricci Dock,Russell Gateway,Hodgkinson Hub,Tiptree Hub,Pytheas Port,Kier Exchange"
+ },
+ {
+ "name": "Aoniu",
+ "pos_x": 77.75,
+ "pos_y": -151.84375,
+ "pos_z": 15.03125,
+ "stations": "Bohr Enterprise,Derekas Prospect,Artsutanov Terminal"
+ },
+ {
+ "name": "Aornum",
+ "pos_x": 7.5625,
+ "pos_y": -34.90625,
+ "pos_z": 31.625,
+ "stations": "Agassiz City,Kelly Terminal,Hardwick Orbital,Manakov Port,Ham Port,Vaucanson Terminal,Kazantsev Palace,Pausch Station"
+ },
+ {
+ "name": "Aowica",
+ "pos_x": 116.84375,
+ "pos_y": -55.84375,
+ "pos_z": 84.46875,
+ "stations": "Gonnessiat Dock,Takahashi Platform,Roe Platform,Reed Observatory"
+ },
+ {
+ "name": "Aowicha",
+ "pos_x": -118.0625,
+ "pos_y": 46.125,
+ "pos_z": -71.53125,
+ "stations": "Ostrander Relay,Altman Ring"
+ },
+ {
+ "name": "Aowindui",
+ "pos_x": 160.21875,
+ "pos_y": -43.0625,
+ "pos_z": -7.34375,
+ "stations": "Hirayama Survey,Arnold Hub,Phillips Settlement,Dashiell's Inheritance,Menezes Landing"
+ },
+ {
+ "name": "Apach",
+ "pos_x": 178.53125,
+ "pos_y": -134.375,
+ "pos_z": 36.6875,
+ "stations": "Hussey Port,Dhawan Vision"
+ },
+ {
+ "name": "Apachan",
+ "pos_x": 85.75,
+ "pos_y": -40.34375,
+ "pos_z": -125.96875,
+ "stations": "Aitken Landing,Hamilton Survey"
+ },
+ {
+ "name": "Apachanus",
+ "pos_x": -79.125,
+ "pos_y": -135.09375,
+ "pos_z": 21.21875,
+ "stations": "Pavlou Point,Dias Settlement"
+ },
+ {
+ "name": "Apachi",
+ "pos_x": -25.4375,
+ "pos_y": 137,
+ "pos_z": -96.5,
+ "stations": "Brand Landing,Manning Survey"
+ },
+ {
+ "name": "Apacnada",
+ "pos_x": -69.8125,
+ "pos_y": -104.125,
+ "pos_z": 82.75,
+ "stations": "Monge's Inheritance,Vinge Platform,Butler Settlement"
+ },
+ {
+ "name": "Apacnalis",
+ "pos_x": 134.09375,
+ "pos_y": 121.4375,
+ "pos_z": 21.75,
+ "stations": "Land Station,Gidzenko Barracks,Buckey Enterprise,Ivanov Platform"
+ },
+ {
+ "name": "Apadecavi",
+ "pos_x": -5.25,
+ "pos_y": 5.59375,
+ "pos_z": -117.5625,
+ "stations": "Fontenay City,Nelder City,Bixby Enterprise,Barnes Ring,Coats Arsenal"
+ },
+ {
+ "name": "Apademak",
+ "pos_x": -57.8125,
+ "pos_y": -13.65625,
+ "pos_z": -134.65625,
+ "stations": "Scott Orbital,Tenn Park,Pohl Orbital"
+ },
+ {
+ "name": "Apadipinook",
+ "pos_x": 53.6875,
+ "pos_y": -66.53125,
+ "pos_z": 8.84375,
+ "stations": "Deslandres Installation,Clute Settlement,Watson Reach,Condit Holdings,Peters Port"
+ },
+ {
+ "name": "Apala",
+ "pos_x": -59.5625,
+ "pos_y": 31.65625,
+ "pos_z": -23.3125,
+ "stations": "Wilson City,Bose Bastion,Popper Port,Walker Port,Compton Observatory"
+ },
+ {
+ "name": "Apalaina",
+ "pos_x": 105.5625,
+ "pos_y": -52.375,
+ "pos_z": -80.28125,
+ "stations": "Miletus Terminal,Nemere Settlement"
+ },
+ {
+ "name": "Apalar",
+ "pos_x": -28.3125,
+ "pos_y": -77,
+ "pos_z": 56,
+ "stations": "Svavarsson Terminal,Walker Penal colony,Ryan Landing"
+ },
+ {
+ "name": "Apaline",
+ "pos_x": 2.59375,
+ "pos_y": 90.03125,
+ "pos_z": -161.75,
+ "stations": "Greenland Platform,Wallace Mines,Steinmuller Prospect"
+ },
+ {
+ "name": "Apalok",
+ "pos_x": 19.875,
+ "pos_y": -101.96875,
+ "pos_z": -79.59375,
+ "stations": "Vela Orbital,Hahn Terminal"
+ },
+ {
+ "name": "Apam Napat",
+ "pos_x": 10.09375,
+ "pos_y": -90.46875,
+ "pos_z": -0.71875,
+ "stations": "Majida,Sunyaev Beacon,Fan Platform,Langley Hub"
+ },
+ {
+ "name": "Aparctias",
+ "pos_x": 25.1875,
+ "pos_y": -56.375,
+ "pos_z": 22.90625,
+ "stations": "Gooch's Progress,Hancock Refinery,Almadrava,Readdy's Progress"
+ },
+ {
+ "name": "Apathaam",
+ "pos_x": 89.03125,
+ "pos_y": -102.625,
+ "pos_z": 20.875,
+ "stations": "Reamy Dock"
+ },
+ {
+ "name": "Apaticultu",
+ "pos_x": 38.78125,
+ "pos_y": -30.25,
+ "pos_z": -83.65625,
+ "stations": "Salak Dock"
+ },
+ {
+ "name": "Apatugans",
+ "pos_x": 57.9375,
+ "pos_y": -240.84375,
+ "pos_z": 40.6875,
+ "stations": "Foster Depot"
+ },
+ {
+ "name": "Aphra",
+ "pos_x": -68.53125,
+ "pos_y": -4.375,
+ "pos_z": 60.3125,
+ "stations": "Beckman Terminal,McKay Beacon,Aldiss Depot"
+ },
+ {
+ "name": "Apiaca",
+ "pos_x": -15.46875,
+ "pos_y": -191.53125,
+ "pos_z": 131.28125,
+ "stations": "Dominique Point,Rabinowitz Stop"
+ },
+ {
+ "name": "Apian Yi",
+ "pos_x": 64.625,
+ "pos_y": -102.03125,
+ "pos_z": -78.65625,
+ "stations": "Fowler Platform"
+ },
+ {
+ "name": "Apik",
+ "pos_x": -0.03125,
+ "pos_y": -18.21875,
+ "pos_z": 83.0625,
+ "stations": "Porsche Port,Sharman City,Bose Dock,Bugrov Landing,Bradshaw Keep"
+ },
+ {
+ "name": "Apikuna",
+ "pos_x": 20.625,
+ "pos_y": -166.75,
+ "pos_z": 123.375,
+ "stations": "Shea Vision,Giunta Lab"
+ },
+ {
+ "name": "Apinayimas",
+ "pos_x": -19.03125,
+ "pos_y": 41.34375,
+ "pos_z": -102.875,
+ "stations": "Soddy Hangar"
+ },
+ {
+ "name": "Apino",
+ "pos_x": 100.625,
+ "pos_y": -73.34375,
+ "pos_z": 58.53125,
+ "stations": "Fleming Mines"
+ },
+ {
+ "name": "Apinouphis",
+ "pos_x": -102.0625,
+ "pos_y": 12.4375,
+ "pos_z": -45.625,
+ "stations": "Pippin Terminal"
+ },
+ {
+ "name": "Apinyalivun",
+ "pos_x": 80.6875,
+ "pos_y": 12.625,
+ "pos_z": -103.875,
+ "stations": "Parazynski Terminal,Hopkins Port"
+ },
+ {
+ "name": "Apiru",
+ "pos_x": -46.53125,
+ "pos_y": -161.6875,
+ "pos_z": -36.21875,
+ "stations": "Malchiodi Dock,Stjepan Seljan Dock"
+ },
+ {
+ "name": "Apis",
+ "pos_x": 186.125,
+ "pos_y": -155.6875,
+ "pos_z": 85.9375,
+ "stations": "Challis Mine"
+ },
+ {
+ "name": "Apishna",
+ "pos_x": 45.5625,
+ "pos_y": -106.375,
+ "pos_z": -22.09375,
+ "stations": "Hamuy Port,Ackerman Penal colony,Dominique Terminal,Smyth Survey"
+ },
+ {
+ "name": "Apisina",
+ "pos_x": -23.28125,
+ "pos_y": 78.875,
+ "pos_z": -137.6875,
+ "stations": "Tsibliyev Legacy,Grego Installation"
+ },
+ {
+ "name": "Apisni",
+ "pos_x": 120.78125,
+ "pos_y": -68.90625,
+ "pos_z": 48.4375,
+ "stations": "Hansteen Hub,Tanaka Orbital,Mizuno Terminal,Ilyushin Dock,Perrine Orbital,Coande Port,Stabenow Vision,Chasles Lab,Chorel Penal colony,Ashbrook Dock,Pierce Base"
+ },
+ {
+ "name": "Apisnik",
+ "pos_x": -72.9375,
+ "pos_y": -8.8125,
+ "pos_z": 149.9375,
+ "stations": "Garn Mines"
+ },
+ {
+ "name": "Apisulfr",
+ "pos_x": 103.8125,
+ "pos_y": -82.40625,
+ "pos_z": 82.21875,
+ "stations": "Foden Depot,Lilienthal Port,Johri Gateway,Wingrove's Inheritance,Birkhoff Installation"
+ },
+ {
+ "name": "Apollo",
+ "pos_x": -11.21875,
+ "pos_y": -27.4375,
+ "pos_z": -45.59375,
+ "stations": "Payson Plant,Reisman Station,Fulton Landing,Gooch Base"
+ },
+ {
+ "name": "Apotanites",
+ "pos_x": 102.25,
+ "pos_y": -159.75,
+ "pos_z": 188.21875,
+ "stations": "Galois Terminal,Westerhout Beacon"
+ },
+ {
+ "name": "Apotec",
+ "pos_x": -122.96875,
+ "pos_y": 110.15625,
+ "pos_z": -9.375,
+ "stations": "Chorel Legacy"
+ },
+ {
+ "name": "Apotenile",
+ "pos_x": -22.25,
+ "pos_y": -102.625,
+ "pos_z": -77.875,
+ "stations": "McIntyre Port,Tilley Station,Cousteau Survey,Bernard Terminal"
+ },
+ {
+ "name": "Apoya",
+ "pos_x": 4.90625,
+ "pos_y": -107.125,
+ "pos_z": -122.8125,
+ "stations": "Regiomontanus Settlement,Normand Platform,Parry Landing,Tarski's Progress"
+ },
+ {
+ "name": "Apoyas",
+ "pos_x": 84.53125,
+ "pos_y": -50.6875,
+ "pos_z": 26.1875,
+ "stations": "Markov Vision,Tomita Port,Vess Dock,Bliss Hangar"
+ },
+ {
+ "name": "Apoyot",
+ "pos_x": 58.875,
+ "pos_y": -5.84375,
+ "pos_z": 102.09375,
+ "stations": "Makarov Ring"
+ },
+ {
+ "name": "Apoyota",
+ "pos_x": -33.6875,
+ "pos_y": -4.34375,
+ "pos_z": 61.375,
+ "stations": "Flint Station,Hahn Relay"
+ },
+ {
+ "name": "Apsaras",
+ "pos_x": 130.28125,
+ "pos_y": -223.9375,
+ "pos_z": 64.34375,
+ "stations": "Jansky Orbital,Lockwood Survey"
+ },
+ {
+ "name": "Apsarillici",
+ "pos_x": -42.6875,
+ "pos_y": -159.53125,
+ "pos_z": -50.15625,
+ "stations": "Korolyov Terminal,Kemurdzhian Vision,Herbig Relay,Shoemaker Orbital"
+ },
+ {
+ "name": "Apsarre",
+ "pos_x": 42.71875,
+ "pos_y": -128.71875,
+ "pos_z": 112.8125,
+ "stations": "Dedman Survey,Altuna Settlement"
+ },
+ {
+ "name": "Apsu",
+ "pos_x": 10.125,
+ "pos_y": -91.84375,
+ "pos_z": 51.75,
+ "stations": "Dickinson Hangar,Tusi Terminal,Emshwiller Town"
+ },
+ {
+ "name": "Apterisha",
+ "pos_x": 71.46875,
+ "pos_y": -96.9375,
+ "pos_z": 101.625,
+ "stations": "Shen Port"
+ },
+ {
+ "name": "Apternuteri",
+ "pos_x": -63.65625,
+ "pos_y": 145.75,
+ "pos_z": 13.46875,
+ "stations": "Boas Mines,Watson Colony"
+ },
+ {
+ "name": "Aptet",
+ "pos_x": -82.09375,
+ "pos_y": 26.375,
+ "pos_z": 59.28125,
+ "stations": "Torricelli Port"
+ },
+ {
+ "name": "Apteti",
+ "pos_x": -127.59375,
+ "pos_y": -91.71875,
+ "pos_z": 27.25,
+ "stations": "Becquerel Port,Young Base,Sturckow Ring,Henry Park,Abel Landing"
+ },
+ {
+ "name": "Apura",
+ "pos_x": 41.8125,
+ "pos_y": 57.34375,
+ "pos_z": 5.40625,
+ "stations": "Wiener Port,Fozard Port,Fuca Dock,Comer Terminal,Kozlov Vision,Chalker Ring,Piccard Gateway,Howard Vision,Danvers Installation,Maclaurin Depot,Lee Installation"
+ },
+ {
+ "name": "Apurarai",
+ "pos_x": -46.9375,
+ "pos_y": -77.03125,
+ "pos_z": 66.65625,
+ "stations": "Watts Platform,Kessel Platform"
+ },
+ {
+ "name": "Apuri",
+ "pos_x": -35.96875,
+ "pos_y": -137.3125,
+ "pos_z": 60.40625,
+ "stations": "Phillips Station"
+ },
+ {
+ "name": "Apurineph",
+ "pos_x": -3.6875,
+ "pos_y": -164.3125,
+ "pos_z": -34.5,
+ "stations": "Alexandrov Vision,Popov Landing"
+ },
+ {
+ "name": "Apuris",
+ "pos_x": 18.40625,
+ "pos_y": -43.15625,
+ "pos_z": -149.65625,
+ "stations": "Vespucci Stop"
+ },
+ {
+ "name": "Apurnarsu",
+ "pos_x": 53.21875,
+ "pos_y": -82,
+ "pos_z": -0.6875,
+ "stations": "Hariot Dock,Johnson Prospect,Kohoutek Installation"
+ },
+ {
+ "name": "Apuru",
+ "pos_x": 88.375,
+ "pos_y": -154.9375,
+ "pos_z": 10.59375,
+ "stations": "Weaver Mine"
+ },
+ {
+ "name": "Apurui",
+ "pos_x": 96.21875,
+ "pos_y": -75.3125,
+ "pos_z": 81.4375,
+ "stations": "Lange Gateway,Dugan Gateway,Hereford City,Cook Hub,Marcy Gateway,Burckhardt Relay,Bridger Horizons,Lyulka Hub,Byrd Vista"
+ },
+ {
+ "name": "Apuruk",
+ "pos_x": 81.84375,
+ "pos_y": -114.5625,
+ "pos_z": -50.3125,
+ "stations": "Wood Dock,Esclangon Bastion,Thesiger Horizons"
+ },
+ {
+ "name": "Apurusia",
+ "pos_x": 8.46875,
+ "pos_y": -172,
+ "pos_z": -20.28125,
+ "stations": "Herbig Station,Cowper's Inheritance,Lane Survey,Leydenfrost Orbital"
+ },
+ {
+ "name": "Aquixing",
+ "pos_x": 30.90625,
+ "pos_y": -153.46875,
+ "pos_z": -83.5625,
+ "stations": "Kraft Point,Cuffey Vista"
+ },
+ {
+ "name": "AR Lacertae",
+ "pos_x": -137.3125,
+ "pos_y": -20.1875,
+ "pos_z": -14.4375,
+ "stations": "Buffett Port,Kregel Hub,Faris Orbital,Sladek Beacon,Nobel Hub"
+ },
+ {
+ "name": "Arabaka",
+ "pos_x": 127.125,
+ "pos_y": -47.3125,
+ "pos_z": 36.65625,
+ "stations": "Antonov Outpost,Chawla Point"
+ },
+ {
+ "name": "Arabakti",
+ "pos_x": -18.125,
+ "pos_y": -23.25,
+ "pos_z": 85.15625,
+ "stations": "Afanasyev Plant,Edison Dock"
+ },
+ {
+ "name": "Arabakuala",
+ "pos_x": 98.9375,
+ "pos_y": 83.84375,
+ "pos_z": 110.15625,
+ "stations": "Thesiger Penal colony,Rotsler Penal colony,Nikitin Base"
+ },
+ {
+ "name": "Arabana",
+ "pos_x": 17.625,
+ "pos_y": -188.1875,
+ "pos_z": 3.5,
+ "stations": "Fuller Beacon"
+ },
+ {
+ "name": "Arabarente",
+ "pos_x": 135.78125,
+ "pos_y": -53.5625,
+ "pos_z": -22.125,
+ "stations": "Macedo Refinery,Serling Terminal,Mechain Holdings"
+ },
+ {
+ "name": "Arabari",
+ "pos_x": -100.3125,
+ "pos_y": -0.65625,
+ "pos_z": -123.21875,
+ "stations": "Bailey Enterprise,Wyndham Installation,Godel Relay"
+ },
+ {
+ "name": "Arabayati",
+ "pos_x": 59.9375,
+ "pos_y": 9.5625,
+ "pos_z": 126.71875,
+ "stations": "Resnick Settlement,Elder Settlement"
+ },
+ {
+ "name": "Arabela",
+ "pos_x": 32.125,
+ "pos_y": -78.75,
+ "pos_z": 98.5625,
+ "stations": "Stromgren Port"
+ },
+ {
+ "name": "Arabh",
+ "pos_x": -73.625,
+ "pos_y": 64.46875,
+ "pos_z": 11.96875,
+ "stations": "Gentle Reach,Precourt Enterprise,Doctorow Orbital,Cleve Orbital,Heceta Market,Normand Dock,Weston Base,Hovgaard Hub"
+ },
+ {
+ "name": "Arabha",
+ "pos_x": -138.09375,
+ "pos_y": -15.84375,
+ "pos_z": 37.28125,
+ "stations": "Morgan Colony,Leibniz Relay"
+ },
+ {
+ "name": "Arabhairiwa",
+ "pos_x": 79.15625,
+ "pos_y": 87.5,
+ "pos_z": 114.90625,
+ "stations": "Schottky Dock,Carr's Folly"
+ },
+ {
+ "name": "Arabrakale",
+ "pos_x": -99.3125,
+ "pos_y": -111.09375,
+ "pos_z": -19.65625,
+ "stations": "Moisuc Terminal,Conway Orbital"
+ },
+ {
+ "name": "Arabrigur",
+ "pos_x": -0.5,
+ "pos_y": -149.15625,
+ "pos_z": 86.96875,
+ "stations": "May Station"
+ },
+ {
+ "name": "Arabrini",
+ "pos_x": 56.1875,
+ "pos_y": -78.5625,
+ "pos_z": -52.25,
+ "stations": "Wells Platform,Varley Relay"
+ },
+ {
+ "name": "Arabru",
+ "pos_x": 85.84375,
+ "pos_y": -163.5625,
+ "pos_z": 87.96875,
+ "stations": "Samos Survey,Yanai Port,McCaffrey Vision"
+ },
+ {
+ "name": "Aracae",
+ "pos_x": -2.9375,
+ "pos_y": 99.75,
+ "pos_z": 96.09375,
+ "stations": "Weinbaum Settlement,Stiegler Point"
+ },
+ {
+ "name": "Aracame",
+ "pos_x": 42.5,
+ "pos_y": 56.53125,
+ "pos_z": -103.0625,
+ "stations": "Ford Point,Ansari Horizons"
+ },
+ {
+ "name": "Aracente",
+ "pos_x": 90.28125,
+ "pos_y": -44.3125,
+ "pos_z": 54.75,
+ "stations": "Bahcall Settlement"
+ },
+ {
+ "name": "Aracenth",
+ "pos_x": 147.125,
+ "pos_y": -207.9375,
+ "pos_z": -2.53125,
+ "stations": "Arend Mines,Takahashi Port"
+ },
+ {
+ "name": "Aracenus",
+ "pos_x": 111.3125,
+ "pos_y": -65.875,
+ "pos_z": 68,
+ "stations": "Chernykh Hub,Mason Port,Fraunhofer Ring,Hayashi Vision,Ingstad Keep,Ejigu Dock,Hoffmeister Station,Jenkins Station,Ziegler Base,Fadlan Terminal,Alvarez de Pineda's Inheritance"
+ },
+ {
+ "name": "Arachi Szu",
+ "pos_x": 60.15625,
+ "pos_y": -231.21875,
+ "pos_z": 5.625,
+ "stations": "Bowen Terminal"
+ },
+ {
+ "name": "Aracinicnii",
+ "pos_x": 70.96875,
+ "pos_y": 80.1875,
+ "pos_z": -11.6875,
+ "stations": "Yano Co-operative,Hannu Enterprise"
+ },
+ {
+ "name": "Arakak",
+ "pos_x": 41.4375,
+ "pos_y": -40.8125,
+ "pos_z": -104.75,
+ "stations": "Burnet's Claim,Chu Settlement"
+ },
+ {
+ "name": "Arakandza",
+ "pos_x": 115.15625,
+ "pos_y": -121.25,
+ "pos_z": 167.34375,
+ "stations": "Siegel Beacon,Trujillo Reach"
+ },
+ {
+ "name": "Arakang",
+ "pos_x": 48.8125,
+ "pos_y": 3,
+ "pos_z": -168,
+ "stations": "Wilson Mines,Witt Legacy,Tall Beacon"
+ },
+ {
+ "name": "Arakapajo",
+ "pos_x": 144.34375,
+ "pos_y": -73.375,
+ "pos_z": -12.28125,
+ "stations": "Libeskind Hub,Bailey Enterprise,Crossfield City,Tange Gateway,Hansteen Terminal,Bauman Plant,Stephan Port,Fabricius Colony"
+ },
+ {
+ "name": "Arakates",
+ "pos_x": 167,
+ "pos_y": -186.5625,
+ "pos_z": -44.125,
+ "stations": "Garrido Reach"
+ },
+ {
+ "name": "Araki",
+ "pos_x": -179.15625,
+ "pos_y": 38.53125,
+ "pos_z": 57.40625,
+ "stations": "Perga Terminal,Grigson Landing"
+ },
+ {
+ "name": "Arakwa",
+ "pos_x": 97.0625,
+ "pos_y": -225.1875,
+ "pos_z": -2.6875,
+ "stations": "Alexandria Point,Bassford's Claim"
+ },
+ {
+ "name": "Arakwibut",
+ "pos_x": -107.40625,
+ "pos_y": -59.96875,
+ "pos_z": 102.15625,
+ "stations": "Bolotov Gateway,Wilcutt Ring,Leestma Mines"
+ },
+ {
+ "name": "Araman",
+ "pos_x": 160.4375,
+ "pos_y": -85.28125,
+ "pos_z": 37.0625,
+ "stations": "Pacheco's Folly,Hereford Installation,Reeves Settlement,Giacobini Platform,Milne Base"
+ },
+ {
+ "name": "Arambe",
+ "pos_x": -44.53125,
+ "pos_y": 80.59375,
+ "pos_z": 54.28125,
+ "stations": "Pohl Terminal,Sterling Base"
+ },
+ {
+ "name": "Aramo",
+ "pos_x": 123.0625,
+ "pos_y": 32.28125,
+ "pos_z": -143.375,
+ "stations": "Evans Outpost,Williams Platform,Cartmill Vision"
+ },
+ {
+ "name": "Aramorans",
+ "pos_x": -115.84375,
+ "pos_y": 60.75,
+ "pos_z": 36.8125,
+ "stations": "Stafford Enterprise,Fuglesang Station,Gardner Port,Cockrell Terminal,Satcher Gateway,Schlegel Terminal,Aleksandrov Gateway,Arthur Landing,Schilling Depot,Amundsen Holdings,Kelleam Landing,Verne Terminal,Humphreys Vision"
+ },
+ {
+ "name": "Aramorians",
+ "pos_x": 70.34375,
+ "pos_y": 32.03125,
+ "pos_z": -20.21875,
+ "stations": "Avogadro Plant"
+ },
+ {
+ "name": "Aramu",
+ "pos_x": -101.3125,
+ "pos_y": -41.875,
+ "pos_z": 34.5625,
+ "stations": "Terry Prospect,Malcolm Installation"
+ },
+ {
+ "name": "Aramzahd",
+ "pos_x": 66.46875,
+ "pos_y": -144.46875,
+ "pos_z": 29.875,
+ "stations": "Norman - Mavis's Bingo Palace,Koishikawa Dock,Wyndham Point"
+ },
+ {
+ "name": "Aranadan",
+ "pos_x": -1.1875,
+ "pos_y": 26.21875,
+ "pos_z": 159.5625,
+ "stations": "Poindexter Enterprise,Fourneyron Park,Szilard Hub,Ozanne Barracks"
+ },
+ {
+ "name": "Aranbarahun",
+ "pos_x": 78.96875,
+ "pos_y": 15.625,
+ "pos_z": 65.8125,
+ "stations": "Ross Orbital,Burstein Horizons,Barnes Terminal,Silverberg Station,Drake Refinery,Lamarck Palace,Culpeper Survey,Narvaez's Claim,Roberts Orbital,Grijalva Hub,Brandenstein Installation,Pavlou Port,van Vogt Ring,Paulo da Gama Hub,Gessi Gateway,Melroy Reach"
+ },
+ {
+ "name": "Arangorii",
+ "pos_x": 19.15625,
+ "pos_y": -10.375,
+ "pos_z": 15.40625,
+ "stations": "Kaku Terminal,Maudslay Dock,Watt Enterprise,Zhen Hub,Shonin Station,Leibniz Orbital,Ziljak Colony,Houtman Exchange"
+ },
+ {
+ "name": "Aranka",
+ "pos_x": -49.40625,
+ "pos_y": -44.34375,
+ "pos_z": -62.90625,
+ "stations": "Caselberg Depot,Walker Port,Townshend Gateway,Bishop Lab"
+ },
+ {
+ "name": "Aranner",
+ "pos_x": -52.75,
+ "pos_y": -157.59375,
+ "pos_z": 1.28125,
+ "stations": "Thorne Landing,Fernao do Po Landing,Banno Platform"
+ },
+ {
+ "name": "Arannos",
+ "pos_x": 97.5,
+ "pos_y": -25,
+ "pos_z": -1.34375,
+ "stations": "Weyn Relay,Sekelj Installation"
+ },
+ {
+ "name": "Arany",
+ "pos_x": -49.21875,
+ "pos_y": 56.8125,
+ "pos_z": 83.34375,
+ "stations": "Ford Terminal,Garriott Dock,Harvey Terminal,Richards Beacon,Alexander Lab"
+ },
+ {
+ "name": "Arapa",
+ "pos_x": 119.4375,
+ "pos_y": -98.0625,
+ "pos_z": -7.09375,
+ "stations": "Hoffmeister Mines"
+ },
+ {
+ "name": "Arapahoma",
+ "pos_x": -98.03125,
+ "pos_y": -34.875,
+ "pos_z": 99.53125,
+ "stations": "Bunch Terminal,Stafford Beacon,Mitchell Orbital,Sladek Horizons"
+ },
+ {
+ "name": "Arapani",
+ "pos_x": 119.84375,
+ "pos_y": -208.1875,
+ "pos_z": 123.8125,
+ "stations": "Gill Mines,Dominique Dock,Adams Point"
+ },
+ {
+ "name": "Arapathaka",
+ "pos_x": 114.28125,
+ "pos_y": -48.3125,
+ "pos_z": 18.40625,
+ "stations": "Cartan's Inheritance,Robert Prospect,Burbidge Platform"
+ },
+ {
+ "name": "Arapi",
+ "pos_x": 60.875,
+ "pos_y": 98.78125,
+ "pos_z": 46.15625,
+ "stations": "Feoktistov Terminal,Perga's Inheritance,Coats' Claim,RenenBellot Works"
+ },
+ {
+ "name": "Arapipi",
+ "pos_x": 100.625,
+ "pos_y": -192.4375,
+ "pos_z": 54.71875,
+ "stations": "Bohrmann Prospect,Coande Colony,Naylor Ring"
+ },
+ {
+ "name": "Arara",
+ "pos_x": -97.34375,
+ "pos_y": -41.625,
+ "pos_z": -51.9375,
+ "stations": "Szilard Ring,Kuhn Dock"
+ },
+ {
+ "name": "Arare",
+ "pos_x": 28.125,
+ "pos_y": -40.5625,
+ "pos_z": 30.78125,
+ "stations": "Noriega Port"
+ },
+ {
+ "name": "Ararex",
+ "pos_x": 113.21875,
+ "pos_y": 83.34375,
+ "pos_z": -102.25,
+ "stations": "Rozhdestvensky Dock,Oluwafemi Port,Budrys Enterprise,Aitken Enterprise"
+ },
+ {
+ "name": "Arasan",
+ "pos_x": 195.53125,
+ "pos_y": -121.0625,
+ "pos_z": 84.53125,
+ "stations": "Kirk Gateway,Lubbock Settlement,Bereznyak Gateway,Hottot Penal colony,Kippax Prospect"
+ },
+ {
+ "name": "Arasee",
+ "pos_x": 109.28125,
+ "pos_y": 18.21875,
+ "pos_z": -31.5625,
+ "stations": "Hunt Terminal,Shaver Settlement,Windt Settlement"
+ },
+ {
+ "name": "Arasiri",
+ "pos_x": 159.6875,
+ "pos_y": -156.09375,
+ "pos_z": -18.8125,
+ "stations": "Derekas Vision,Bok Vision,Vyssotsky Vision"
+ },
+ {
+ "name": "Aravaci",
+ "pos_x": 41.625,
+ "pos_y": 18.40625,
+ "pos_z": -78.875,
+ "stations": "Sommerfeld Mine,Alexandria Terminal"
+ },
+ {
+ "name": "Aravaledi",
+ "pos_x": -31.9375,
+ "pos_y": 44.65625,
+ "pos_z": 56.34375,
+ "stations": "Thorne Holdings,Paez Depot,Flade Hub,Varthema Laboratory"
+ },
+ {
+ "name": "Aravuni",
+ "pos_x": 135.5625,
+ "pos_y": 31.6875,
+ "pos_z": -69,
+ "stations": "Gamow City,Payton Depot,Meredith Keep"
+ },
+ {
+ "name": "Arawamina",
+ "pos_x": 120.15625,
+ "pos_y": 38.34375,
+ "pos_z": -61.6875,
+ "stations": "Culbertson Base,Heck's Claim"
+ },
+ {
+ "name": "Arawan",
+ "pos_x": 145.8125,
+ "pos_y": -5.6875,
+ "pos_z": -22.59375,
+ "stations": "Morgan Beacon"
+ },
+ {
+ "name": "Arawas",
+ "pos_x": -151.03125,
+ "pos_y": 30.125,
+ "pos_z": 23.65625,
+ "stations": "Tennyson d'Eyncourt Refinery,May City"
+ },
+ {
+ "name": "Arawegali",
+ "pos_x": -113.34375,
+ "pos_y": 30.125,
+ "pos_z": 107.59375,
+ "stations": "Moffitt Point"
+ },
+ {
+ "name": "Arawere",
+ "pos_x": -43.71875,
+ "pos_y": -101.59375,
+ "pos_z": 24.90625,
+ "stations": "Korolyov Hub,Tanaka Gateway"
+ },
+ {
+ "name": "Arawn",
+ "pos_x": -5,
+ "pos_y": -157.25,
+ "pos_z": 39.59375,
+ "stations": "Shute Settlement"
+ },
+ {
+ "name": "Arawot",
+ "pos_x": 69.90625,
+ "pos_y": -28.875,
+ "pos_z": 113.3125,
+ "stations": "Shunn Lab,Moffitt Relay"
+ },
+ {
+ "name": "Arawotyan",
+ "pos_x": 27.90625,
+ "pos_y": 137.5,
+ "pos_z": -37.03125,
+ "stations": "Whitney Dock,Overmyer Port,Merbold Terminal,Meucci Vision,Santos Base"
+ },
+ {
+ "name": "Arawotyas",
+ "pos_x": -84.5625,
+ "pos_y": -114,
+ "pos_z": 86.96875,
+ "stations": "Sheffield Port,Eschbach Point,Tiptree Silo"
+ },
+ {
+ "name": "Arawoyn",
+ "pos_x": -60.21875,
+ "pos_y": -43.90625,
+ "pos_z": -43.21875,
+ "stations": "Gunn Relay,Weiss Base"
+ },
+ {
+ "name": "Arbuda",
+ "pos_x": -34.09375,
+ "pos_y": 26.21875,
+ "pos_z": -5.53125,
+ "stations": "Hawking Station,Novitski Oasis"
+ },
+ {
+ "name": "Arcturus",
+ "pos_x": -3.53125,
+ "pos_y": 34.15625,
+ "pos_z": 12.96875,
+ "stations": "Brennan Depot,Hunziker Enterprise,Sarmiento de Gamboa Market,Alison City,Hooperport,Fort Harrison,Abel Plant,Singh Orbiter,Salgari Vista"
+ },
+ {
+ "name": "Ardh",
+ "pos_x": 99.5625,
+ "pos_y": 2.46875,
+ "pos_z": 1.78125,
+ "stations": "Nusslein-Volhard Platform"
+ },
+ {
+ "name": "Ardhin",
+ "pos_x": -33.25,
+ "pos_y": -4.15625,
+ "pos_z": -163.75,
+ "stations": "Kozeyev's Claim,Vittori Mines,Cabrera Camp"
+ },
+ {
+ "name": "Ardhri",
+ "pos_x": -108.34375,
+ "pos_y": 37.125,
+ "pos_z": -78.96875,
+ "stations": "Beekman Orbital,Cook Horizons,Wafer Horizons"
+ },
+ {
+ "name": "Ardiaei",
+ "pos_x": -118.09375,
+ "pos_y": -50.34375,
+ "pos_z": 54.09375,
+ "stations": "Rangarajan Platform"
+ },
+ {
+ "name": "Ardians",
+ "pos_x": 60.15625,
+ "pos_y": -80.59375,
+ "pos_z": 137.84375,
+ "stations": "Kidinnu Orbital,Arago Gateway,Matthews Port,Monge Holdings,Laumer's Progress,Pannekoek Enterprise"
+ },
+ {
+ "name": "Ardin",
+ "pos_x": 105.40625,
+ "pos_y": -224.96875,
+ "pos_z": 7.40625,
+ "stations": "Anastase Perrotin's Claim,Foerster Colony,Peary Penal colony"
+ },
+ {
+ "name": "Ardraj",
+ "pos_x": -125.78125,
+ "pos_y": 10.3125,
+ "pos_z": -8.375,
+ "stations": "Wallace Orbital,Smith Relay,Padalka Penal colony"
+ },
+ {
+ "name": "Arebatec",
+ "pos_x": -20.59375,
+ "pos_y": 49.15625,
+ "pos_z": 142.1875,
+ "stations": "Moran Beacon"
+ },
+ {
+ "name": "Arebeghe",
+ "pos_x": 160.8125,
+ "pos_y": -144.21875,
+ "pos_z": 38.90625,
+ "stations": "Barbuy's Claim,Bond Colony"
+ },
+ {
+ "name": "Arebelatja",
+ "pos_x": -63.03125,
+ "pos_y": 48.25,
+ "pos_z": -122.40625,
+ "stations": "Redi Camp,Gutierrez Hub,Banks Prospect"
+ },
+ {
+ "name": "Arecoya",
+ "pos_x": -4.75,
+ "pos_y": -190.0625,
+ "pos_z": -18.15625,
+ "stations": "Albategnius Dock,Kojima Enterprise"
+ },
+ {
+ "name": "Arek",
+ "pos_x": 21.59375,
+ "pos_y": 61.6875,
+ "pos_z": 113.09375,
+ "stations": "Alexander Port,Moon Station,Gold Hub,Thompson Terminal,Brooks Point,Herbert Station"
+ },
+ {
+ "name": "Areklici",
+ "pos_x": 84.75,
+ "pos_y": 22.125,
+ "pos_z": -68.53125,
+ "stations": "Heinlein Dock,Cole Silo,Maire Vision"
+ },
+ {
+ "name": "Arevaci",
+ "pos_x": -161.53125,
+ "pos_y": 77.875,
+ "pos_z": 88.25,
+ "stations": "Coulter Base"
+ },
+ {
+ "name": "Arevakimos",
+ "pos_x": 29.4375,
+ "pos_y": -64.96875,
+ "pos_z": 89.6875,
+ "stations": "Roth Port,Digges Horizons,Beaumont Penal colony,Hampson Dock,Schwabe Hub,Truman Port,Finlay-Freundlich Hub,Kidd Prospect,Nijland Vision,Sitterly City,Green's Inheritance,Harawi Hub,Bracewell Hub,Cuffey Vision,Schomburg Hub"
+ },
+ {
+ "name": "Arevas",
+ "pos_x": 74.71875,
+ "pos_y": -40.375,
+ "pos_z": 124.125,
+ "stations": "Neujmin City,Hirayama Port"
+ },
+ {
+ "name": "Arevat",
+ "pos_x": 40.65625,
+ "pos_y": -204.125,
+ "pos_z": 71.6875,
+ "stations": "Baliunas Vision,Gernsback Prospect,Gaultier de Varennes Relay"
+ },
+ {
+ "name": "Arexack",
+ "pos_x": 44.96875,
+ "pos_y": -80.53125,
+ "pos_z": -7.21875,
+ "stations": "Fortress Gordon,Rance Station,Farmer City,Lopez City,Macquorn Rankine Colony"
+ },
+ {
+ "name": "Arexe",
+ "pos_x": 69.375,
+ "pos_y": 38.90625,
+ "pos_z": 78.0625,
+ "stations": "Janes Horizons,Vinge Keep"
+ },
+ {
+ "name": "Argel",
+ "pos_x": 111.625,
+ "pos_y": -71.40625,
+ "pos_z": 126.9375,
+ "stations": "H. G. Wells Relay,Staus Horizons,Godwin Point"
+ },
+ {
+ "name": "Argen",
+ "pos_x": -23.375,
+ "pos_y": -153.5625,
+ "pos_z": 31.84375,
+ "stations": "Schaumasse Station,Russell Vision,Schroter Colony"
+ },
+ {
+ "name": "Argeno",
+ "pos_x": -4.5,
+ "pos_y": -15.53125,
+ "pos_z": 153.28125,
+ "stations": "Lichtenberg Platform"
+ },
+ {
+ "name": "Argenoi",
+ "pos_x": 139.625,
+ "pos_y": -10.9375,
+ "pos_z": 101.875,
+ "stations": "McArthur Stop,Poincare's Inheritance"
+ },
+ {
+ "name": "Argestes",
+ "pos_x": 21.78125,
+ "pos_y": -20.15625,
+ "pos_z": -58.9375,
+ "stations": "von Bellingshausen Station,Alexandria's Claim,la Cosa Enterprise,Soper Vision,Anthony de la Roche Vision,Burton Station,MacLeod Enterprise,Smith Dock,Wolfe Port,Friesner Orbital,Clement Terminal,Blackwell Landing"
+ },
+ {
+ "name": "Argetlamh",
+ "pos_x": 85.75,
+ "pos_y": -170.6875,
+ "pos_z": 74.9375,
+ "stations": "Meuron Port,Baade Arsenal,Hirayama Ring,Lalande Terminal"
+ },
+ {
+ "name": "Arhul",
+ "pos_x": -39,
+ "pos_y": -52.375,
+ "pos_z": 119.875,
+ "stations": "Schweickart Orbital,Haisheng Settlement,Rangarajan Mine"
+ },
+ {
+ "name": "Ari He",
+ "pos_x": -158.09375,
+ "pos_y": 72.15625,
+ "pos_z": 1.25,
+ "stations": "Stanley Dock"
+ },
+ {
+ "name": "Ari Hesa",
+ "pos_x": -116.9375,
+ "pos_y": 11.5625,
+ "pos_z": 46.0625,
+ "stations": "Atwater City,Wilmore Hub,Gemar Station,Shatalov City,Hieb Dock"
+ },
+ {
+ "name": "Ariameni",
+ "pos_x": -42.03125,
+ "pos_y": 35.53125,
+ "pos_z": -143.875,
+ "stations": "Landsteiner City,Patrick Enterprise,Norton Installation,Tilman Relay"
+ },
+ {
+ "name": "Ariatia",
+ "pos_x": -4.0625,
+ "pos_y": -130.6875,
+ "pos_z": -96.15625,
+ "stations": "Lovelace Orbital,Gooch Ring,Anderson Installation"
+ },
+ {
+ "name": "Arietis Sector AG-O b6-2",
+ "pos_x": -81.53125,
+ "pos_y": -101.65625,
+ "pos_z": -76.46875,
+ "stations": "Barth Reach,Wundt Base"
+ },
+ {
+ "name": "Arietis Sector AI-H a11-3",
+ "pos_x": -113.125,
+ "pos_y": -25.875,
+ "pos_z": -103.375,
+ "stations": "Gabriel Installation"
+ },
+ {
+ "name": "Arietis Sector AQ-P b5-0",
+ "pos_x": -81.34375,
+ "pos_y": -140.65625,
+ "pos_z": -101.25,
+ "stations": "Larson Relay"
+ },
+ {
+ "name": "Arietis Sector AQ-P b5-2",
+ "pos_x": -82.25,
+ "pos_y": -129.46875,
+ "pos_z": -97.0625,
+ "stations": "Baudin Installation"
+ },
+ {
+ "name": "Arietis Sector BQ-Y d114",
+ "pos_x": -115.34375,
+ "pos_y": -12.5,
+ "pos_z": -137.125,
+ "stations": "Cenker Exchange"
+ },
+ {
+ "name": "Arietis Sector BV-Y c7",
+ "pos_x": -61.8125,
+ "pos_y": -27.65625,
+ "pos_z": -160.71875,
+ "stations": "Oltion Terminal"
+ },
+ {
+ "name": "Arietis Sector CG-X c1-20",
+ "pos_x": -88.6875,
+ "pos_y": -12.71875,
+ "pos_z": -128.78125,
+ "stations": "Armstrong's Inheritance,Kent Installation"
+ },
+ {
+ "name": "Arietis Sector CQ-Y d60",
+ "pos_x": -42.09375,
+ "pos_y": -6.40625,
+ "pos_z": -112.9375,
+ "stations": "Cochrane Works,Hillary Keep"
+ },
+ {
+ "name": "Arietis Sector DB-W b2-0",
+ "pos_x": -55.78125,
+ "pos_y": -6.59375,
+ "pos_z": -163.46875,
+ "stations": "Greenleaf Settlement,Harding Installation"
+ },
+ {
+ "name": "Arietis Sector DB-X c1-3",
+ "pos_x": -133.0625,
+ "pos_y": -59.125,
+ "pos_z": -108.5,
+ "stations": "Wiener Exchange"
+ },
+ {
+ "name": "Arietis Sector DB-X c1-4",
+ "pos_x": -130.8125,
+ "pos_y": -56.625,
+ "pos_z": -112.3125,
+ "stations": "Eisenstein's Folly"
+ },
+ {
+ "name": "Arietis Sector DB-X c1-5",
+ "pos_x": -118.90625,
+ "pos_y": -64.96875,
+ "pos_z": -107.8125,
+ "stations": "Alvarez de Pineda Settlement"
+ },
+ {
+ "name": "Arietis Sector DB-X c1-6",
+ "pos_x": -114.03125,
+ "pos_y": -60.3125,
+ "pos_z": -114.78125,
+ "stations": "Offutt Survey"
+ },
+ {
+ "name": "Arietis Sector DL-Y d79",
+ "pos_x": -116.6875,
+ "pos_y": -40.0625,
+ "pos_z": -119.40625,
+ "stations": "Caryanda's Claim"
+ },
+ {
+ "name": "Arietis Sector DL-Y d95",
+ "pos_x": -101.40625,
+ "pos_y": -84.9375,
+ "pos_z": -126.375,
+ "stations": "Sellings Relay,Alexander Base"
+ },
+ {
+ "name": "Arietis Sector DQ-Y c16",
+ "pos_x": -28.125,
+ "pos_y": -79.8125,
+ "pos_z": -157.03125,
+ "stations": "Spinrad Prospect,Ziemianski's Inheritance"
+ },
+ {
+ "name": "Arietis Sector DQ-Y c18",
+ "pos_x": -27.28125,
+ "pos_y": -71.9375,
+ "pos_z": -168.9375,
+ "stations": "Soukup Depot,Mendel Depot"
+ },
+ {
+ "name": "Arietis Sector DQ-Y c19",
+ "pos_x": -52.46875,
+ "pos_y": -87.09375,
+ "pos_z": -167.6875,
+ "stations": "Salak Prospect"
+ },
+ {
+ "name": "Arietis Sector EQ-Y c17",
+ "pos_x": -24.53125,
+ "pos_y": -87.6875,
+ "pos_z": -166.4375,
+ "stations": "Martins Observatory"
+ },
+ {
+ "name": "Arietis Sector FW-W c1-16",
+ "pos_x": -139.34375,
+ "pos_y": -81.3125,
+ "pos_z": -106.4375,
+ "stations": "Norgay Colony"
+ },
+ {
+ "name": "Arietis Sector FW-W c1-18",
+ "pos_x": -139.25,
+ "pos_y": -79.5625,
+ "pos_z": -112.78125,
+ "stations": "Steinmuller's Pride"
+ },
+ {
+ "name": "Arietis Sector FW-W c1-19",
+ "pos_x": -141.59375,
+ "pos_y": -78.34375,
+ "pos_z": -107.03125,
+ "stations": "Yolen's Claim"
+ },
+ {
+ "name": "Arietis Sector FW-W c1-4",
+ "pos_x": -135.71875,
+ "pos_y": -77.96875,
+ "pos_z": -114.1875,
+ "stations": "Hendel Settlement"
+ },
+ {
+ "name": "Arietis Sector GG-Y d63",
+ "pos_x": -52.125,
+ "pos_y": -120.5625,
+ "pos_z": -126.65625,
+ "stations": "Dickson Refinery"
+ },
+ {
+ "name": "Arietis Sector GW-W c1-13",
+ "pos_x": -99.28125,
+ "pos_y": -94.3125,
+ "pos_z": -121.625,
+ "stations": "Miletus' Inheritance,Rondon Keep"
+ },
+ {
+ "name": "Arietis Sector GW-W c1-4",
+ "pos_x": -87.5625,
+ "pos_y": -97.625,
+ "pos_z": -138.125,
+ "stations": "Blaylock's Progress,Shepherd Landing"
+ },
+ {
+ "name": "Arietis Sector GW-W c1-6",
+ "pos_x": -87.5,
+ "pos_y": -92.25,
+ "pos_z": -108.5,
+ "stations": "Hume Arsenal"
+ },
+ {
+ "name": "Arietis Sector GW-W c1-8",
+ "pos_x": -78.1875,
+ "pos_y": -81.90625,
+ "pos_z": -117.78125,
+ "stations": "Brothers Plant,Davis Beacon"
+ },
+ {
+ "name": "Arietis Sector IR-V b2-0",
+ "pos_x": -33.78125,
+ "pos_y": -52.71875,
+ "pos_z": -163.875,
+ "stations": "Ray Camp"
+ },
+ {
+ "name": "Arietis Sector JR-W c1-12",
+ "pos_x": -38.28125,
+ "pos_y": -122.28125,
+ "pos_z": -126.15625,
+ "stations": "Bergerac Mines,Janes Settlement"
+ },
+ {
+ "name": "Arietis Sector JR-W c1-13",
+ "pos_x": -29.25,
+ "pos_y": -137.125,
+ "pos_z": -129.8125,
+ "stations": "Penn Settlement"
+ },
+ {
+ "name": "Arietis Sector JR-W c1-15",
+ "pos_x": -28.03125,
+ "pos_y": -132.59375,
+ "pos_z": -140.375,
+ "stations": "Ford Installation"
+ },
+ {
+ "name": "Arietis Sector JR-W c1-16",
+ "pos_x": -25.84375,
+ "pos_y": -134.5625,
+ "pos_z": -144.71875,
+ "stations": "d'Eyncourt Keep,Savorgnan de Brazza's Inheritance"
+ },
+ {
+ "name": "Arietis Sector JR-W c1-19",
+ "pos_x": -48.625,
+ "pos_y": -119.71875,
+ "pos_z": -132.90625,
+ "stations": "Barnaby's Progress"
+ },
+ {
+ "name": "Arietis Sector JR-W c1-20",
+ "pos_x": -47.65625,
+ "pos_y": -115.90625,
+ "pos_z": -136.28125,
+ "stations": "Serrao's Pride"
+ },
+ {
+ "name": "Arietis Sector KC-V c2-20",
+ "pos_x": -95.78125,
+ "pos_y": -80.96875,
+ "pos_z": -79.78125,
+ "stations": "Ponce de Leon Terminal,Weyl Reach"
+ },
+ {
+ "name": "Arietis Sector KM-W d1-94",
+ "pos_x": -57.5,
+ "pos_y": -132.96875,
+ "pos_z": -96.59375,
+ "stations": "Dickson's Claim,Beekman Prospect"
+ },
+ {
+ "name": "Arietis Sector LX-U c2-12",
+ "pos_x": -107.65625,
+ "pos_y": -120.25,
+ "pos_z": -78.84375,
+ "stations": "Robinson Landing,Goldstein Works,Hennepin Holdings"
+ },
+ {
+ "name": "Arietis Sector MX-U c2-18",
+ "pos_x": -87.5625,
+ "pos_y": -139.9375,
+ "pos_z": -97,
+ "stations": "Wollheim Horizons"
+ },
+ {
+ "name": "Arietis Sector ND-S b4-2",
+ "pos_x": -103.4375,
+ "pos_y": -63.96875,
+ "pos_z": -114.71875,
+ "stations": "Farkas Vision"
+ },
+ {
+ "name": "Arietis Sector NT-Q b5-4",
+ "pos_x": -104.90625,
+ "pos_y": -15.5625,
+ "pos_z": -98.46875,
+ "stations": "Kurland Beacon,Bailey Installation"
+ },
+ {
+ "name": "Arietis Sector ON-T b3-3",
+ "pos_x": -75.5,
+ "pos_y": -93,
+ "pos_z": -131.46875,
+ "stations": "Phillips Holdings"
+ },
+ {
+ "name": "Arietis Sector ON-T b3-4",
+ "pos_x": -77.90625,
+ "pos_y": -94.375,
+ "pos_z": -135.28125,
+ "stations": "Paulo da Gama Horizons,Coulomb's Folly"
+ },
+ {
+ "name": "Arietis Sector OS-T b3-0",
+ "pos_x": -39.5625,
+ "pos_y": -81.59375,
+ "pos_z": -144.15625,
+ "stations": "Arnold Horizons"
+ },
+ {
+ "name": "Arietis Sector ST-R b4-1",
+ "pos_x": -70.46875,
+ "pos_y": -89.15625,
+ "pos_z": -107.21875,
+ "stations": "Stross Market"
+ },
+ {
+ "name": "Arietis Sector VZ-P b5-5",
+ "pos_x": -100.125,
+ "pos_y": -95.5625,
+ "pos_z": -94.96875,
+ "stations": "Nehsi Landing,Harrison Silo"
+ },
+ {
+ "name": "Arietis Sector WK-M a8-0",
+ "pos_x": -54.4375,
+ "pos_y": -45,
+ "pos_z": -125.46875,
+ "stations": "Hobaugh Depot,Tolstoi Vision"
+ },
+ {
+ "name": "Arietis Sector WK-O b6-5",
+ "pos_x": -118.9375,
+ "pos_y": -81.6875,
+ "pos_z": -77.96875,
+ "stations": "Plante Vision,Svavarsson Enterprise"
+ },
+ {
+ "name": "Arietis Sector XE-Z b4",
+ "pos_x": -98.78125,
+ "pos_y": -54.03125,
+ "pos_z": -199.6875,
+ "stations": "PRE Logistics Support Beta"
+ },
+ {
+ "name": "Arietis Sector YZ-Y c4",
+ "pos_x": -97.3125,
+ "pos_y": -15,
+ "pos_z": -149.125,
+ "stations": "Hartlib Enterprise"
+ },
+ {
+ "name": "Arietis Sector ZF-O b6-1",
+ "pos_x": -89.53125,
+ "pos_y": -102.4375,
+ "pos_z": -75.4375,
+ "stations": "Penal Ship Y-32"
+ },
+ {
+ "name": "Arietis Sector ZZ-Y c12",
+ "pos_x": -46.96875,
+ "pos_y": -6.15625,
+ "pos_z": -151.875,
+ "stations": "Capek Point"
+ },
+ {
+ "name": "Ariga",
+ "pos_x": -83.46875,
+ "pos_y": -96.40625,
+ "pos_z": -23.34375,
+ "stations": "Moisuc's Claim"
+ },
+ {
+ "name": "Arige",
+ "pos_x": 163.1875,
+ "pos_y": -59.71875,
+ "pos_z": -14.625,
+ "stations": "Herbig Vision,Beliaev Landing,Jefferies Prospect"
+ },
+ {
+ "name": "Arigens",
+ "pos_x": 1.75,
+ "pos_y": -15.6875,
+ "pos_z": 69.25,
+ "stations": "Ordway Ring,Riazuddin Depot,Dummer Orbital,Cannon's Progress"
+ },
+ {
+ "name": "Arikara",
+ "pos_x": 106.25,
+ "pos_y": -156.5,
+ "pos_z": 43.90625,
+ "stations": "Bennett Gateway,Kuchemann Installation,Sternbach Hub,Karman Port,Fleming Terminal,Lalande Station,Robson Base,Kotelnikov Ring,de Kamp Gateway,Carroll City,Pimi Terminal,Mozhaysky's Progress,Sellings Holdings,Hansteen Port,Bradley Depot"
+ },
+ {
+ "name": "Arikaras",
+ "pos_x": -80.5625,
+ "pos_y": -54.34375,
+ "pos_z": -63.46875,
+ "stations": "Vonnegut Beacon,Lander Mine"
+ },
+ {
+ "name": "Ariku",
+ "pos_x": 104.6875,
+ "pos_y": -74.40625,
+ "pos_z": 131.15625,
+ "stations": "Roth Port,Pook Hub,Samos Beacon"
+ },
+ {
+ "name": "Arikudiil",
+ "pos_x": 111.8125,
+ "pos_y": -222.9375,
+ "pos_z": 85.84375,
+ "stations": "Henderson Stop,Parkinson's Claim,Gulyaev Base"
+ },
+ {
+ "name": "Arimavante",
+ "pos_x": 55.21875,
+ "pos_y": -164.53125,
+ "pos_z": 95.84375,
+ "stations": "Soto's Inheritance,Lyot Dock,Bickel City,Lagadha Keep"
+ },
+ {
+ "name": "Arimil",
+ "pos_x": -68.28125,
+ "pos_y": -30.0625,
+ "pos_z": -56.84375,
+ "stations": "Pippin Dock,Artyukhin Keep"
+ },
+ {
+ "name": "Ariminimi",
+ "pos_x": -23,
+ "pos_y": 6.78125,
+ "pos_z": -116,
+ "stations": "Rusch Prospect,Stein Survey,Clark Legacy"
+ },
+ {
+ "name": "Arimpox",
+ "pos_x": -39.90625,
+ "pos_y": 109.5625,
+ "pos_z": 10.40625,
+ "stations": "Linenger Ring,Morgan Station,Agnesi Dock,Bessemer Terminal,Velazquez Station,Pierres Enterprise,Pournelle Relay,Matthews Beacon"
+ },
+ {
+ "name": "Arinack",
+ "pos_x": 11.9375,
+ "pos_y": -43.03125,
+ "pos_z": -22.90625,
+ "stations": "Nicolet City,Kier Penal colony,Burke Horizons,Wiener Enterprise"
+ },
+ {
+ "name": "Arinas",
+ "pos_x": 117.125,
+ "pos_y": -182.28125,
+ "pos_z": 12.21875,
+ "stations": "Nojiri Settlement,Brashear Platform,Delporte Works"
+ },
+ {
+ "name": "Arindan",
+ "pos_x": 20.375,
+ "pos_y": -45.09375,
+ "pos_z": 109.53125,
+ "stations": "Krigstein Mine,Goodman Settlement"
+ },
+ {
+ "name": "Arine",
+ "pos_x": -143.6875,
+ "pos_y": 40.6875,
+ "pos_z": -77.5,
+ "stations": "Wescott Terminal,Poncelet Terminal,Boe Dock,Foda Orbital"
+ },
+ {
+ "name": "Arinman",
+ "pos_x": -4,
+ "pos_y": 77.75,
+ "pos_z": -138.3125,
+ "stations": "Laplace Port"
+ },
+ {
+ "name": "Arinna",
+ "pos_x": -50.75,
+ "pos_y": -1.90625,
+ "pos_z": -21.75,
+ "stations": "Pavlov Dock,Engle Orbital,Nordenskiold Stop"
+ },
+ {
+ "name": "Aritimi",
+ "pos_x": -64.65625,
+ "pos_y": 31.0625,
+ "pos_z": 19.875,
+ "stations": "Armstrong Dock,Huygens Port,Padalka Orbital,Griggs Hub,Campbell's Pride,Aguirre Hub"
+ },
+ {
+ "name": "Arjung",
+ "pos_x": 15.53125,
+ "pos_y": -100.1875,
+ "pos_z": -87.28125,
+ "stations": "Hiyya Orbital,Fallows Ring,Lee Depot,al-Kashi Orbital"
+ },
+ {
+ "name": "Arkab Posterior",
+ "pos_x": 13.625,
+ "pos_y": -56.09375,
+ "pos_z": 121.09375,
+ "stations": "Akers' Folly,Keyes Mines"
+ },
+ {
+ "name": "Arm",
+ "pos_x": -60.53125,
+ "pos_y": -100.375,
+ "pos_z": 111.0625,
+ "stations": "Bohr Station,Byrd Survey,Vela Port,Macquorn Rankine Terminal,Payne Installation"
+ },
+ {
+ "name": "Armo",
+ "pos_x": 69.3125,
+ "pos_y": -174.1875,
+ "pos_z": 66.46875,
+ "stations": "Lagerkvist's Progress,Stromgren Survey,Abe Depot"
+ },
+ {
+ "name": "Armod",
+ "pos_x": 146.875,
+ "pos_y": 23.375,
+ "pos_z": 12.0625,
+ "stations": "Khayyam Orbital,Nielsen Penal colony"
+ },
+ {
+ "name": "Armodiang",
+ "pos_x": 84.75,
+ "pos_y": -159.21875,
+ "pos_z": -25.3125,
+ "stations": "Ryle Dock,Nomen Hub,Libeskind Port,Nilson Settlement,Kopff Installation"
+ },
+ {
+ "name": "Armotai",
+ "pos_x": 59.15625,
+ "pos_y": 22.53125,
+ "pos_z": 109.375,
+ "stations": "Pribylov Landing"
+ },
+ {
+ "name": "Arnais",
+ "pos_x": 40.03125,
+ "pos_y": 21.46875,
+ "pos_z": -123.625,
+ "stations": "Laliberte Ring,Wrangell Orbital,Adams Gateway,Sadi Carnot Hub,Veblen Survey,Hogan Point,Hurston Ring"
+ },
+ {
+ "name": "Arnaq",
+ "pos_x": 62.78125,
+ "pos_y": -35.5,
+ "pos_z": -75.5625,
+ "stations": "Thuot Port"
+ },
+ {
+ "name": "Arnaqu",
+ "pos_x": -93.71875,
+ "pos_y": -34.25,
+ "pos_z": 77.65625,
+ "stations": "Richards Port,Wellman Oasis"
+ },
+ {
+ "name": "Arnaqui",
+ "pos_x": -24.03125,
+ "pos_y": -56.84375,
+ "pos_z": 98.625,
+ "stations": "Heaviside Hub,Bunch Colony,Conklin Station"
+ },
+ {
+ "name": "Arne Naom",
+ "pos_x": -83.78125,
+ "pos_y": 32.40625,
+ "pos_z": 13.90625,
+ "stations": "Haipeng Gateway"
+ },
+ {
+ "name": "Arnemil",
+ "pos_x": -38.5,
+ "pos_y": -2.875,
+ "pos_z": -67.8125,
+ "stations": "Clervoy Hub,Barsanti Landing,Buffett Beacon"
+ },
+ {
+ "name": "Arnga",
+ "pos_x": -46.1875,
+ "pos_y": -105.375,
+ "pos_z": 6.5625,
+ "stations": "Charnas Camp"
+ },
+ {
+ "name": "Arngi",
+ "pos_x": 11.71875,
+ "pos_y": -72.125,
+ "pos_z": 4.78125,
+ "stations": "Novitski Mines,Otto Vision"
+ },
+ {
+ "name": "Arngin",
+ "pos_x": 97.5,
+ "pos_y": -114.78125,
+ "pos_z": 40.375,
+ "stations": "Martiniere Survey,Ziegel Colony,Elst Port"
+ },
+ {
+ "name": "Arouca",
+ "pos_x": 104.96875,
+ "pos_y": -6.53125,
+ "pos_z": -4.40625,
+ "stations": "Shipton Orbital,Borisenko's Folly,Siegel Hub,Wilkes Installation"
+ },
+ {
+ "name": "Arque",
+ "pos_x": 66.5,
+ "pos_y": 38.0625,
+ "pos_z": 61.125,
+ "stations": "Abel Laboratory,Austen Town Station,Baird Gateway,Smith Prospect"
+ },
+ {
+ "name": "Arro Kop",
+ "pos_x": 100.3125,
+ "pos_y": 38.71875,
+ "pos_z": 94.0625,
+ "stations": "Svavarsson City,Shaikh Hub"
+ },
+ {
+ "name": "Arro Na",
+ "pos_x": 117.46875,
+ "pos_y": 19.8125,
+ "pos_z": -4.78125,
+ "stations": "Brady Station,Townshend Horizons,Thuot Gateway,Gell-Mann Oasis,Lopez de Haro Installation"
+ },
+ {
+ "name": "Arro Naga",
+ "pos_x": 49.375,
+ "pos_y": 6.1875,
+ "pos_z": 33.09375,
+ "stations": "Gillekens Gateway,Shepherd Gateway"
+ },
+ {
+ "name": "Artemis",
+ "pos_x": 14.28125,
+ "pos_y": -63.1875,
+ "pos_z": -24.875,
+ "stations": "Freeholm,Laphrian Shipyard,Burckhardt Station,Suri Base"
+ },
+ {
+ "name": "Arth",
+ "pos_x": 36.375,
+ "pos_y": -15.40625,
+ "pos_z": 32.9375,
+ "stations": "Midgeley Dock"
+ },
+ {
+ "name": "Artha",
+ "pos_x": -168.1875,
+ "pos_y": 69.5625,
+ "pos_z": -7.03125,
+ "stations": "Herbert Platform,Sterling Vision,Barjavel Beacon,Heaviside Enterprise"
+ },
+ {
+ "name": "Arthaiyati",
+ "pos_x": 117.5,
+ "pos_y": 8.0625,
+ "pos_z": 104.03125,
+ "stations": "Sevastyanov Enterprise,Hermaszewski Station,Diesel Survey,Carter Prospect"
+ },
+ {
+ "name": "Arthel",
+ "pos_x": -23.90625,
+ "pos_y": -210.71875,
+ "pos_z": 8.3125,
+ "stations": "Kepler Legacy,Kowal Arsenal"
+ },
+ {
+ "name": "Arthinn",
+ "pos_x": -31.40625,
+ "pos_y": 120.375,
+ "pos_z": 121.03125,
+ "stations": "Moffitt Survey,Bisson Arena"
+ },
+ {
+ "name": "Arti",
+ "pos_x": 144.78125,
+ "pos_y": -121.09375,
+ "pos_z": -41.75,
+ "stations": "Luyten Survey,Ashbrook Enterprise"
+ },
+ {
+ "name": "Artii",
+ "pos_x": -81.40625,
+ "pos_y": 127.46875,
+ "pos_z": -67.21875,
+ "stations": "Macgregor Terminal,Clauss Landing,Leckie Refinery"
+ },
+ {
+ "name": "Artume",
+ "pos_x": -35.21875,
+ "pos_y": -42.625,
+ "pos_z": -49.65625,
+ "stations": "Sanctuary,Ferdie,Jenkinson Terminal,Bennett Gateway"
+ },
+ {
+ "name": "Artumes",
+ "pos_x": -10.875,
+ "pos_y": 61.5625,
+ "pos_z": -40.9375,
+ "stations": "McArthur Orbital,Fibonacci's Inheritance,Junlong Settlement"
+ },
+ {
+ "name": "Aruagea",
+ "pos_x": -90.6875,
+ "pos_y": 32.375,
+ "pos_z": 36.71875,
+ "stations": "Hornby Colony"
+ },
+ {
+ "name": "Aruan",
+ "pos_x": -85.78125,
+ "pos_y": -117.78125,
+ "pos_z": -1.40625,
+ "stations": "Quimby Platform"
+ },
+ {
+ "name": "Aruaycuru",
+ "pos_x": 143.28125,
+ "pos_y": -121.5,
+ "pos_z": -14.25,
+ "stations": "Smoot Point,Thiele Mine"
+ },
+ {
+ "name": "Arug",
+ "pos_x": 30.53125,
+ "pos_y": -253.875,
+ "pos_z": 52.65625,
+ "stations": "Jansky Vision"
+ },
+ {
+ "name": "Arugbal",
+ "pos_x": -86.4375,
+ "pos_y": -116.15625,
+ "pos_z": -65.71875,
+ "stations": "Bryant Platform,Meaney Dock,Haarsma Colony"
+ },
+ {
+ "name": "Arugbatz",
+ "pos_x": -123.15625,
+ "pos_y": 118.65625,
+ "pos_z": -63.1875,
+ "stations": "Francisco de Almeida Landing,Klein Port,Pike Landing,Rothman's Progress,Sawyer Beacon"
+ },
+ {
+ "name": "Arugh",
+ "pos_x": 124.125,
+ "pos_y": -123.65625,
+ "pos_z": 6.59375,
+ "stations": "Walotsky Terminal,Petlyakov Ring,Oren Gateway,Menzies Depot,Mitchell Prospect"
+ },
+ {
+ "name": "Arugu",
+ "pos_x": 79.6875,
+ "pos_y": -103.5625,
+ "pos_z": 77.21875,
+ "stations": "Jung Colony,Hale Depot,Rosenberger Colony"
+ },
+ {
+ "name": "Arugua",
+ "pos_x": 96.03125,
+ "pos_y": -171.46875,
+ "pos_z": 36.25,
+ "stations": "Herzog Orbital,Bowell Terminal,Noon Base,Giclas Hub"
+ },
+ {
+ "name": "Arugundji",
+ "pos_x": 38.78125,
+ "pos_y": -120.125,
+ "pos_z": -59.90625,
+ "stations": "Eggleton Terminal,Pordenone Gateway,Laing Refinery,Adelman Ring,Wafa Ring,Vogel Station,Phillips Dock,Kuchner Port,Hiyya Dock"
+ },
+ {
+ "name": "Arun",
+ "pos_x": 105.25,
+ "pos_y": -46.625,
+ "pos_z": -10.40625,
+ "stations": "Fabricius Mine,Sabine Installation"
+ },
+ {
+ "name": "Arunab",
+ "pos_x": 95.9375,
+ "pos_y": -102.03125,
+ "pos_z": 110.3125,
+ "stations": "Bauman Vision,Whitford Gateway,Skiff Orbital"
+ },
+ {
+ "name": "Arundji",
+ "pos_x": 31.5,
+ "pos_y": 71.25,
+ "pos_z": 140.5625,
+ "stations": "Sakers Penal colony,Caselberg Barracks"
+ },
+ {
+ "name": "Arungu",
+ "pos_x": 119.65625,
+ "pos_y": -135.78125,
+ "pos_z": 123.625,
+ "stations": "Leberecht Tempel Mines,Schmidt Prospect,Bayley Works"
+ },
+ {
+ "name": "Arunmi",
+ "pos_x": -102.4375,
+ "pos_y": 48.59375,
+ "pos_z": 76.03125,
+ "stations": "Gagnan Vision,Cernan Camp,Shumil Keep"
+ },
+ {
+ "name": "Aruntei",
+ "pos_x": 144.34375,
+ "pos_y": -144,
+ "pos_z": -63.90625,
+ "stations": "Piano Settlement,Ayton Innes Vision,Bartini Vision,Clarke Legacy"
+ },
+ {
+ "name": "Aruru",
+ "pos_x": 17.6875,
+ "pos_y": -13.8125,
+ "pos_z": -43.78125,
+ "stations": "Ford Station,Clark Port"
+ },
+ {
+ "name": "Arverda",
+ "pos_x": -71.34375,
+ "pos_y": -163.6875,
+ "pos_z": 7.71875,
+ "stations": "Levi-Montalcini Enterprise,Noguchi Dock,Darwin Station,McDaniel's Progress"
+ },
+ {
+ "name": "Arverni",
+ "pos_x": -59,
+ "pos_y": -72.90625,
+ "pos_z": 153.15625,
+ "stations": "Padalka Orbital,Cseszneky Holdings"
+ },
+ {
+ "name": "Arvetii",
+ "pos_x": 158.65625,
+ "pos_y": -130.0625,
+ "pos_z": -70.1875,
+ "stations": "McAuley Bastion,Argelander Platform,Kresak Port"
+ },
+ {
+ "name": "Arvetit",
+ "pos_x": -14.96875,
+ "pos_y": -109,
+ "pos_z": -66.15625,
+ "stations": "Whitney Orbital,Libby Port,Saberhagen Exchange"
+ },
+ {
+ "name": "Arvirisa",
+ "pos_x": 15.90625,
+ "pos_y": 9.78125,
+ "pos_z": 87.34375,
+ "stations": "Baturin Refinery,Barnaby Settlement,Halsell Refinery"
+ },
+ {
+ "name": "Aryak",
+ "pos_x": 21.84375,
+ "pos_y": 22.96875,
+ "pos_z": 55.75,
+ "stations": "Crouch Hub,Garneau Port,Alten Holdings,Filipchenko Orbital"
+ },
+ {
+ "name": "Aryamin",
+ "pos_x": 19.3125,
+ "pos_y": -77.5,
+ "pos_z": 30.65625,
+ "stations": "Cabral Silo,Kuchner Enterprise,Banno Settlement"
+ },
+ {
+ "name": "Aryanka",
+ "pos_x": -69.4375,
+ "pos_y": -17.59375,
+ "pos_z": 92.46875,
+ "stations": "Alexandrov's Exile"
+ },
+ {
+ "name": "As Igala",
+ "pos_x": 161.5625,
+ "pos_y": -185.0625,
+ "pos_z": -21.53125,
+ "stations": "Lynden-Bell Enterprise,Ogden Silo"
+ },
+ {
+ "name": "As Igalivun",
+ "pos_x": 180.3125,
+ "pos_y": -107.40625,
+ "pos_z": 44.59375,
+ "stations": "Bessel Hub,Whittle Dock,Salgari's Claim"
+ },
+ {
+ "name": "Asana",
+ "pos_x": -64.125,
+ "pos_y": -82.78125,
+ "pos_z": 77.34375,
+ "stations": "Kwolek Works"
+ },
+ {
+ "name": "Asangbal",
+ "pos_x": 83.5625,
+ "pos_y": -22.53125,
+ "pos_z": 147.28125,
+ "stations": "Stewart Base"
+ },
+ {
+ "name": "Asantani",
+ "pos_x": 58.625,
+ "pos_y": -244.625,
+ "pos_z": 17.875,
+ "stations": "Tisserand Colony,Banno Station,Tokubei Holdings"
+ },
+ {
+ "name": "Asase Ya",
+ "pos_x": 96.03125,
+ "pos_y": -126.3125,
+ "pos_z": 21.4375,
+ "stations": "Dreyer Gateway,Hell Port,Aitken Orbital"
+ },
+ {
+ "name": "Asbrua",
+ "pos_x": -105.46875,
+ "pos_y": -38.53125,
+ "pos_z": -13.09375,
+ "stations": "Ray Platform"
+ },
+ {
+ "name": "Ascella",
+ "pos_x": -10.625,
+ "pos_y": -24.28125,
+ "pos_z": 84.125,
+ "stations": "Bayley Arena,Vaugh Ring,Duffy Dock,Usachov Enterprise,Schiltberger Silo"
+ },
+ {
+ "name": "Asege",
+ "pos_x": -5.375,
+ "pos_y": -131.8125,
+ "pos_z": 103.65625,
+ "stations": "Kuo Port"
+ },
+ {
+ "name": "Asegei",
+ "pos_x": 188.03125,
+ "pos_y": -131.0625,
+ "pos_z": 60.8125,
+ "stations": "Haack Reach,Deutsch Installation"
+ },
+ {
+ "name": "Aseges",
+ "pos_x": 138.5625,
+ "pos_y": 79.1875,
+ "pos_z": -5.84375,
+ "stations": "Santarem Beacon"
+ },
+ {
+ "name": "Asegezites",
+ "pos_x": 62.46875,
+ "pos_y": -14.28125,
+ "pos_z": 46.65625,
+ "stations": "Ibold Keep,Zewail Gateway,Peterson Ring,Haxel Dock"
+ },
+ {
+ "name": "Asellus Australis",
+ "pos_x": 52,
+ "pos_y": 71.96875,
+ "pos_z": -95.71875,
+ "stations": "Holden Prospect,Hamilton Mine"
+ },
+ {
+ "name": "Asellus Primus",
+ "pos_x": -23.9375,
+ "pos_y": 40.875,
+ "pos_z": -1.34375,
+ "stations": "Beagle 2 Landing,Foster Research Lab,Baker's Prospect"
+ },
+ {
+ "name": "Asellus Tertius",
+ "pos_x": -79.0625,
+ "pos_y": 142.78125,
+ "pos_z": -7.9375,
+ "stations": "Fernandez Dock,Wolfe Dock,Lindbohm Camp"
+ },
+ {
+ "name": "Aset",
+ "pos_x": 12.1875,
+ "pos_y": 103.75,
+ "pos_z": 100.75,
+ "stations": "Geston Landing,Hand Legacy,Mille Bastion"
+ },
+ {
+ "name": "Asetsa",
+ "pos_x": -24.8125,
+ "pos_y": 96.25,
+ "pos_z": 37.46875,
+ "stations": "Dayuan Gateway,Maybury Ring,Schilling Station"
+ },
+ {
+ "name": "Asetse Eyo",
+ "pos_x": -135.3125,
+ "pos_y": 98.03125,
+ "pos_z": 76.0625,
+ "stations": "Sterling Dock,Isherwood Colony"
+ },
+ {
+ "name": "Asetsi",
+ "pos_x": 100.65625,
+ "pos_y": 35.46875,
+ "pos_z": 58.84375,
+ "stations": "Evans City,Hartlib Hub,Elion Station,O'Connor Port,Melnick Ring,Verne Vision,Cook Hub,Ivanov Enterprise,Grover City,Gann Installation,Froude City,Cayley Terminal"
+ },
+ {
+ "name": "Asga'a",
+ "pos_x": 91,
+ "pos_y": -30.90625,
+ "pos_z": -34.28125,
+ "stations": "Sadi Carnot Platform,Landis Installation"
+ },
+ {
+ "name": "Asgaa",
+ "pos_x": -19.125,
+ "pos_y": 10.6875,
+ "pos_z": 87.34375,
+ "stations": "Wheeler Horizons,Magnus Station,Wiberg Dock"
+ },
+ {
+ "name": "Asgandjin",
+ "pos_x": 80.09375,
+ "pos_y": 55.21875,
+ "pos_z": 17.96875,
+ "stations": "Behnken's Claim,Hoshide Outpost"
+ },
+ {
+ "name": "Asgar",
+ "pos_x": -79.0625,
+ "pos_y": 77.75,
+ "pos_z": -42.84375,
+ "stations": "Windt Station,Wilder Dock"
+ },
+ {
+ "name": "Asgara",
+ "pos_x": 80.875,
+ "pos_y": 4.40625,
+ "pos_z": 77.625,
+ "stations": "Kelly Legacy,Jones Dock"
+ },
+ {
+ "name": "Asgarage",
+ "pos_x": 15.15625,
+ "pos_y": -10.46875,
+ "pos_z": -23.0625,
+ "stations": "Kidman Port,Slonczewski Relay,Low Hub,Low Observatory"
+ },
+ {
+ "name": "Asgariges",
+ "pos_x": -131.0625,
+ "pos_y": 27.21875,
+ "pos_z": -118.1875,
+ "stations": "Gerrold Platform,Shirley Beacon,Guerrero Landing"
+ },
+ {
+ "name": "Asgaru",
+ "pos_x": -125.59375,
+ "pos_y": 0.625,
+ "pos_z": -75.375,
+ "stations": "Surayev Mine"
+ },
+ {
+ "name": "Asha",
+ "pos_x": -65.78125,
+ "pos_y": -25.5,
+ "pos_z": 139.5,
+ "stations": "Cartwright Terminal,Knapp Orbital,Harris Orbital,Disch Depot"
+ },
+ {
+ "name": "Ashandras",
+ "pos_x": -20.1875,
+ "pos_y": -9.0625,
+ "pos_z": -71.40625,
+ "stations": "Bolger Vision,Alpers Refinery"
+ },
+ {
+ "name": "Ashe",
+ "pos_x": -92.21875,
+ "pos_y": 74.21875,
+ "pos_z": 116.09375,
+ "stations": "Tilley Gateway,Aitken Enterprise,Stokes Palace,Borel Settlement"
+ },
+ {
+ "name": "Asherah",
+ "pos_x": 8.53125,
+ "pos_y": 63.0625,
+ "pos_z": 25.125,
+ "stations": "Killough Silo,Holberg Freeport,Francisco de Eliza Prospect"
+ },
+ {
+ "name": "Ashtart",
+ "pos_x": 18.5,
+ "pos_y": 65.84375,
+ "pos_z": 3.28125,
+ "stations": "Nesvadba Orbital,Hinz Hub,Hand Enterprise"
+ },
+ {
+ "name": "Ashvins",
+ "pos_x": 56.09375,
+ "pos_y": 137,
+ "pos_z": -60.8125,
+ "stations": "Gentle Landing,Madsen Landing,Gibson Arsenal"
+ },
+ {
+ "name": "Asinda",
+ "pos_x": -53.6875,
+ "pos_y": -34.96875,
+ "pos_z": 89.46875,
+ "stations": "Tapinas Refinery"
+ },
+ {
+ "name": "Asing Linga",
+ "pos_x": -133.25,
+ "pos_y": 48.28125,
+ "pos_z": 33.09375,
+ "stations": "Grunsfeld Terminal,Salk Enterprise,Aleksandrov Terminal,Birdseye Orbital,Stith Holdings,Vittori's Folly"
+ },
+ {
+ "name": "Asles",
+ "pos_x": 79.53125,
+ "pos_y": 115.0625,
+ "pos_z": -22.9375,
+ "stations": "Pettit Mines"
+ },
+ {
+ "name": "Asletae",
+ "pos_x": 129.78125,
+ "pos_y": 12.71875,
+ "pos_z": -96.59375,
+ "stations": "Lister Dock,Newton Port,Leinster Depot"
+ },
+ {
+ "name": "Asoora",
+ "pos_x": -27.78125,
+ "pos_y": -132.28125,
+ "pos_z": 28.15625,
+ "stations": "Powers Hub,Alden Platform,Clark Camp,Varthema Point"
+ },
+ {
+ "name": "Asooraja",
+ "pos_x": -41.09375,
+ "pos_y": -101.71875,
+ "pos_z": -90.78125,
+ "stations": "Hardy Orbital,Wright Gateway,Dowling Depot"
+ },
+ {
+ "name": "Asoorajara",
+ "pos_x": -98.78125,
+ "pos_y": -99.34375,
+ "pos_z": 22.46875,
+ "stations": "Stableford Hangar,Kennedy Platform"
+ },
+ {
+ "name": "Asoori",
+ "pos_x": 79.90625,
+ "pos_y": 93.40625,
+ "pos_z": -17.15625,
+ "stations": "Carr Hub,Cabana Lab,Utley Barracks"
+ },
+ {
+ "name": "Aspel",
+ "pos_x": -149.875,
+ "pos_y": -14.09375,
+ "pos_z": -54.46875,
+ "stations": "Converse Landing,Rucker Prospect,MacDonald Depot"
+ },
+ {
+ "name": "Aspele",
+ "pos_x": 117.875,
+ "pos_y": -259.25,
+ "pos_z": -22.1875,
+ "stations": "Hyakutake Outpost,Lee Reformatory"
+ },
+ {
+ "name": "Aspelenie",
+ "pos_x": 8.1875,
+ "pos_y": -28.375,
+ "pos_z": -16.84375,
+ "stations": "Boulton Enterprise,Einstein Orbital"
+ },
+ {
+ "name": "Aspelintup",
+ "pos_x": 135.9375,
+ "pos_y": -52.46875,
+ "pos_z": -53.96875,
+ "stations": "Kavandi Gateway,Malpighi Dock,Hieb Orbital,Williamson Beacon,Felice Mine"
+ },
+ {
+ "name": "Asphodel",
+ "pos_x": 48.03125,
+ "pos_y": -117.53125,
+ "pos_z": 12.9375,
+ "stations": "John Irving Station,Saaviks Sanctuary,Brackett Enterprise"
+ },
+ {
+ "name": "Assinda",
+ "pos_x": -70.3125,
+ "pos_y": -118.90625,
+ "pos_z": -112.125,
+ "stations": "Rowley Survey,Hennepin Prospect,Nolan Beacon,Rescue Ship - Hennepin Prospect"
+ },
+ {
+ "name": "Assiniguaye",
+ "pos_x": -130.5625,
+ "pos_y": -2.03125,
+ "pos_z": 41.9375,
+ "stations": "Brothers Dock,Land Prospect"
+ },
+ {
+ "name": "Assiones",
+ "pos_x": 100.90625,
+ "pos_y": -12.96875,
+ "pos_z": 128.59375,
+ "stations": "Buffett Mines"
+ },
+ {
+ "name": "Asterope",
+ "pos_x": -80.15625,
+ "pos_y": -144.09375,
+ "pos_z": -333.375,
+ "stations": "Copernicus Observatory,Rescue Ship - Copernicus Observatory"
+ },
+ {
+ "name": "Astri",
+ "pos_x": -79.9375,
+ "pos_y": -158.3125,
+ "pos_z": 25.875,
+ "stations": "Ponce de Leon Colony,Giles Keep"
+ },
+ {
+ "name": "Astriang",
+ "pos_x": -73.75,
+ "pos_y": 14.34375,
+ "pos_z": -92.59375,
+ "stations": "Kimberlin Vision,Maclaurin Beacon"
+ },
+ {
+ "name": "Astrigond",
+ "pos_x": -42.125,
+ "pos_y": -16.25,
+ "pos_z": 138,
+ "stations": "Reed Orbital,Panshin Refinery"
+ },
+ {
+ "name": "Astrimui",
+ "pos_x": 122.03125,
+ "pos_y": -203.4375,
+ "pos_z": 134.53125,
+ "stations": "Janjetov Holdings,Grushin Survey,Shipton Silo"
+ },
+ {
+ "name": "Asture",
+ "pos_x": -17,
+ "pos_y": -169.3125,
+ "pos_z": 105.65625,
+ "stations": "Ford Installation,Anderson Beacon"
+ },
+ {
+ "name": "Asturindjin",
+ "pos_x": 67.78125,
+ "pos_y": -0.9375,
+ "pos_z": 148.375,
+ "stations": "Melvin Terminal,Scheutz Enterprise,Velazquez Port,Borman Settlement,Shipton Plant"
+ },
+ {
+ "name": "Asura",
+ "pos_x": -9550.28125,
+ "pos_y": -916.65625,
+ "pos_z": 19816.1875,
+ "stations": "Mizuno Dock,Wellington's Claim,Sanctuary"
+ },
+ {
+ "name": "Asurasairu",
+ "pos_x": 24.46875,
+ "pos_y": -8.40625,
+ "pos_z": 89.46875,
+ "stations": "McKay City,Runco Enterprise,Hobaugh Gateway,Boodt Hub"
+ },
+ {
+ "name": "Asurati",
+ "pos_x": 116.65625,
+ "pos_y": -92.1875,
+ "pos_z": 34.5625,
+ "stations": "Sturt's Progress,Kibalchich City,Aoki Dock,Clauss Vision,William Sargent Dock,Smyth Laboratory,Puiseux Station,Coande Dock,Elbakyan Base,Seki Vision,Espenak Port,Whitford Enterprise"
+ },
+ {
+ "name": "Asvien Ek",
+ "pos_x": -19.71875,
+ "pos_y": -100.5625,
+ "pos_z": -45.0625,
+ "stations": "Schoening Prospect,Dolgov Landing,Saavedra's Folly"
+ },
+ {
+ "name": "Asvien Yu",
+ "pos_x": -39.25,
+ "pos_y": -73.375,
+ "pos_z": 145.15625,
+ "stations": "Macgregor Installation,Cady Settlement,Green Installation"
+ },
+ {
+ "name": "Asvieniai",
+ "pos_x": 62.65625,
+ "pos_y": -172.9375,
+ "pos_z": -76.03125,
+ "stations": "Kirkwood Platform,Covington Survey,Witt Point"
+ },
+ {
+ "name": "Asvienses",
+ "pos_x": -36.5625,
+ "pos_y": -112.0625,
+ "pos_z": 34.09375,
+ "stations": "van de Sande Bakhuyzen Silo,Valz Dock,Bracewell Vision,Hynek Installation,Okuni City,Asami City,Sato City,Ross Prospect"
+ },
+ {
+ "name": "Asvincanik",
+ "pos_x": 7.5,
+ "pos_y": -131.53125,
+ "pos_z": -71.875,
+ "stations": "Rankin Platform,Lenthall's Inheritance,Goldreich Dock"
+ },
+ {
+ "name": "Asvincegin",
+ "pos_x": 143.53125,
+ "pos_y": -128.53125,
+ "pos_z": 127.46875,
+ "stations": "Lockyer Orbital,Messerschmitt Orbital"
+ },
+ {
+ "name": "Asvini",
+ "pos_x": 86.90625,
+ "pos_y": -16.09375,
+ "pos_z": -32.8125,
+ "stations": "Bain Platform,Metcalf Enterprise"
+ },
+ {
+ "name": "Asvinia",
+ "pos_x": 70.96875,
+ "pos_y": -30.15625,
+ "pos_z": -61.125,
+ "stations": "Sverdrup Silo,Goeschke Orbital"
+ },
+ {
+ "name": "Asvinici",
+ "pos_x": 2.8125,
+ "pos_y": -90.34375,
+ "pos_z": 80.125,
+ "stations": "Lukyanenko Enterprise,Freycinet Bastion"
+ },
+ {
+ "name": "Asvinnut",
+ "pos_x": -52.78125,
+ "pos_y": -64.4375,
+ "pos_z": 79.3125,
+ "stations": "Cook Station"
+ },
+ {
+ "name": "Atacapa",
+ "pos_x": 23.625,
+ "pos_y": -109.3125,
+ "pos_z": -32.1875,
+ "stations": "van den Hove Prospect,Boss Camp,Hansen Platform,Winthrop Oasis"
+ },
+ {
+ "name": "Ataciangir",
+ "pos_x": 71.125,
+ "pos_y": 51,
+ "pos_z": 128.125,
+ "stations": "Galton Dock,Blalock Orbital,McKay Enterprise,Potagos Mines"
+ },
+ {
+ "name": "Ataco",
+ "pos_x": 95.625,
+ "pos_y": -203.3125,
+ "pos_z": 131.84375,
+ "stations": "McKean Survey,Bond's Exile,Klink Landing"
+ },
+ {
+ "name": "Atacoc",
+ "pos_x": 50.6875,
+ "pos_y": -109.375,
+ "pos_z": 128.65625,
+ "stations": "Amano Port,Ayton Innes Orbital,Mattingly's Progress"
+ },
+ {
+ "name": "Atagat",
+ "pos_x": -1.8125,
+ "pos_y": -11.28125,
+ "pos_z": -29.21875,
+ "stations": "Glashow City,Herzfeld Orbital,Walz Terminal,Stewart Gateway,Lindsey Port,Burgess Holdings,Wang Legacy"
+ },
+ {
+ "name": "Atahingar",
+ "pos_x": -110.15625,
+ "pos_y": -151.59375,
+ "pos_z": -68.28125,
+ "stations": "Oltion Terminal"
+ },
+ {
+ "name": "Ataokomo",
+ "pos_x": 163.6875,
+ "pos_y": -113.4375,
+ "pos_z": 36.53125,
+ "stations": "Hatanaka Works,Shajn Stop,Gloss Landing"
+ },
+ {
+ "name": "Atarapa",
+ "pos_x": 41.5625,
+ "pos_y": 23.5625,
+ "pos_z": -30.5,
+ "stations": "Berliner Station,Gantt Landing,Lind Silo"
+ },
+ {
+ "name": "Atat",
+ "pos_x": 18.1875,
+ "pos_y": 94.53125,
+ "pos_z": -141.09375,
+ "stations": "Lockhart Enterprise,Murdoch Dock,Lyakhov Barracks,Meade's Folly"
+ },
+ {
+ "name": "Atata",
+ "pos_x": -47.09375,
+ "pos_y": -81.90625,
+ "pos_z": 71.4375,
+ "stations": "Zahn Enterprise,Dirichlet Gateway"
+ },
+ {
+ "name": "Atatis",
+ "pos_x": -51.25,
+ "pos_y": 109.0625,
+ "pos_z": -96.8125,
+ "stations": "Abel Terminal,Baxter Works,Buckell Orbital,Drzewiecki Orbital,Crick Prospect"
+ },
+ {
+ "name": "Atatola",
+ "pos_x": 157.46875,
+ "pos_y": -59.5625,
+ "pos_z": 51,
+ "stations": "Qureshi Port"
+ },
+ {
+ "name": "Atayuta",
+ "pos_x": 110.15625,
+ "pos_y": 6.53125,
+ "pos_z": -82.6875,
+ "stations": "Stefansson Platform,Campbell Depot"
+ },
+ {
+ "name": "Aten",
+ "pos_x": -38.125,
+ "pos_y": -32.96875,
+ "pos_z": -17.65625,
+ "stations": "Hale Arsenal,Ramon Depot,Evangelisti Reach,Antonelli Orbital"
+ },
+ {
+ "name": "Ates",
+ "pos_x": 73.875,
+ "pos_y": 59.3125,
+ "pos_z": 146.625,
+ "stations": "Judson Mine"
+ },
+ {
+ "name": "Atese",
+ "pos_x": 76.28125,
+ "pos_y": -179.5625,
+ "pos_z": 203.4375,
+ "stations": "Miyasaka Ring,d'Arrest Station,Boss Station,Butcher Depot"
+ },
+ {
+ "name": "Atfero",
+ "pos_x": 50.03125,
+ "pos_y": -72.875,
+ "pos_z": 46.6875,
+ "stations": "Fernandes' Progress,Wales City,Brothers City,Nijland City,Evans Gateway,Patry Dock,Tisserand Ring,Brooks Camp,Finlay City,Watson Terminal,Dowie Vision,Rasch Arsenal"
+ },
+ {
+ "name": "Athena",
+ "pos_x": 79,
+ "pos_y": -10.1875,
+ "pos_z": 3.21875,
+ "stations": "Darboux Orbital,Delany Laboratory,Powell Terminal,Bohme Hub,Valdes Orbital,Escobar City,Markham City"
+ },
+ {
+ "name": "Athra",
+ "pos_x": -11.53125,
+ "pos_y": -1.5625,
+ "pos_z": -40.53125,
+ "stations": "Comer Depot,Helms Enterprise,Hawley Station,Dobzhansky Orbital,Irwin Port,Baudin Base,Tesla Orbital,Cameron Enterprise,Crowley Landing,Baudry Installation,Froude Arsenal,Mayer Dock"
+ },
+ {
+ "name": "Atins",
+ "pos_x": -117.90625,
+ "pos_y": -12.65625,
+ "pos_z": 115.375,
+ "stations": "Veach Hub,Cheli Station,Bates Hub"
+ },
+ {
+ "name": "Atius",
+ "pos_x": 45.0625,
+ "pos_y": 137.03125,
+ "pos_z": -38.1875,
+ "stations": "Artin Dock,Barnwell's Inheritance,Adams Hangar"
+ },
+ {
+ "name": "Atius Ti",
+ "pos_x": -48.875,
+ "pos_y": -124.09375,
+ "pos_z": -9.125,
+ "stations": "Montanari Vision,Dilworth Outpost"
+ },
+ {
+ "name": "Atiusia",
+ "pos_x": -44,
+ "pos_y": 107.34375,
+ "pos_z": -37.34375,
+ "stations": "Ziljak Port,Sharp Hub,Almagro Ring,Kapp's Progress"
+ },
+ {
+ "name": "Atiusing",
+ "pos_x": 81.21875,
+ "pos_y": 74.9375,
+ "pos_z": -112.03125,
+ "stations": "Wallace Platform"
+ },
+ {
+ "name": "Atjil",
+ "pos_x": 159.9375,
+ "pos_y": -198.28125,
+ "pos_z": 114.84375,
+ "stations": "Danjon Stop,Fedden Stop,Herjulfsson Enterprise"
+ },
+ {
+ "name": "Atjirajan",
+ "pos_x": -106.90625,
+ "pos_y": 53.9375,
+ "pos_z": 39.03125,
+ "stations": "Sakers Hangar,Slade Terminal,Robinson's Inheritance"
+ },
+ {
+ "name": "Atlantis",
+ "pos_x": 65.875,
+ "pos_y": -117.96875,
+ "pos_z": 47.71875,
+ "stations": "Kimura Terminal,Bliss Vision,Ayers City,Comer Arsenal"
+ },
+ {
+ "name": "Atlas",
+ "pos_x": -76.71875,
+ "pos_y": -147.34375,
+ "pos_z": -344.4375,
+ "stations": "Cyllene Orbital,Rescue Ship - Cyllene Orbital"
+ },
+ {
+ "name": "Atora",
+ "pos_x": -148.875,
+ "pos_y": -1.9375,
+ "pos_z": 15.5625,
+ "stations": "Grabe Station,Berners-Lee Colony,Varley Plant"
+ },
+ {
+ "name": "Atorii",
+ "pos_x": -129.15625,
+ "pos_y": -62.71875,
+ "pos_z": 5.84375,
+ "stations": "Butler's Inheritance,Lu Terminal,Anders Terminal,Lousma Port,Alvares Prospect"
+ },
+ {
+ "name": "Atorm",
+ "pos_x": -21.9375,
+ "pos_y": -141.15625,
+ "pos_z": -102.21875,
+ "stations": "Jones Legacy,Stephenson Enterprise"
+ },
+ {
+ "name": "Atrebo",
+ "pos_x": 159.125,
+ "pos_y": -6.5,
+ "pos_z": -22.0625,
+ "stations": "McCarthy's Progress"
+ },
+ {
+ "name": "Atria Bao",
+ "pos_x": -28.46875,
+ "pos_y": -124.21875,
+ "pos_z": -122.625,
+ "stations": "Barnes Survey,Hughes Beacon,Resnick Holdings"
+ },
+ {
+ "name": "Atrigga",
+ "pos_x": 28.90625,
+ "pos_y": -119.0625,
+ "pos_z": 83.71875,
+ "stations": "Matthews Hub,Fleming Terminal,Gessi Point"
+ },
+ {
+ "name": "Atrimih",
+ "pos_x": 104.5,
+ "pos_y": -2.28125,
+ "pos_z": 18.875,
+ "stations": "Polansky Holdings"
+ },
+ {
+ "name": "Atroan",
+ "pos_x": -46.09375,
+ "pos_y": -100.71875,
+ "pos_z": -154.375,
+ "stations": "Cayley Landing"
+ },
+ {
+ "name": "Atroco",
+ "pos_x": -17.28125,
+ "pos_y": -95.75,
+ "pos_z": 37.65625,
+ "stations": "Lasswitz Port,Watts Orbital,Kurland Dock,Hottot Enterprise,Hudson Penal colony"
+ },
+ {
+ "name": "Atron",
+ "pos_x": 101.53125,
+ "pos_y": 41.65625,
+ "pos_z": -144.75,
+ "stations": "Lefschetz's Pride"
+ },
+ {
+ "name": "Atrongo",
+ "pos_x": 19.8125,
+ "pos_y": -119.40625,
+ "pos_z": -67.625,
+ "stations": "Krigstein Enterprise,Dyson Port"
+ },
+ {
+ "name": "Atropos",
+ "pos_x": 41.40625,
+ "pos_y": -29.6875,
+ "pos_z": -27.625,
+ "stations": "vo Market,Walker Settlement,Spedding Orbital,Kepler Orbital,Euler Hub,Dyson Terminal,Pailes Port,Norton Works,Hertz Reach"
+ },
+ {
+ "name": "Atsina",
+ "pos_x": 95.625,
+ "pos_y": -134.46875,
+ "pos_z": 78.125,
+ "stations": "Green Hub,Charlois Station,Szameit Arsenal,Grijalva Vision,Cousin Laboratory"
+ },
+ {
+ "name": "Attacates",
+ "pos_x": 96.625,
+ "pos_y": 1.03125,
+ "pos_z": 103.40625,
+ "stations": "Buckell Ring,Mitchell City,Serre Dock,Theodorsen Survey,Morgan City,Baily Beacon,Wilhelm Hub,Baxter Prospect,Yeliseyev Vista"
+ },
+ {
+ "name": "Attach",
+ "pos_x": -74.34375,
+ "pos_y": -24.25,
+ "pos_z": 49.59375,
+ "stations": "Robinson Gateway,Otiman Terminal"
+ },
+ {
+ "name": "Attada",
+ "pos_x": -162.875,
+ "pos_y": -78.78125,
+ "pos_z": -43.65625,
+ "stations": "Abernathy Point"
+ },
+ {
+ "name": "Atuat",
+ "pos_x": 18.78125,
+ "pos_y": -71.375,
+ "pos_z": 118.875,
+ "stations": "Sabine Orbital,Doctorow Horizons"
+ },
+ {
+ "name": "Atuate",
+ "pos_x": 68.5,
+ "pos_y": 99.46875,
+ "pos_z": 73.625,
+ "stations": "Ising Horizons,Patsayev Outpost"
+ },
+ {
+ "name": "Atuathli",
+ "pos_x": -102.96875,
+ "pos_y": -21.71875,
+ "pos_z": -39.59375,
+ "stations": "Sellers Station"
+ },
+ {
+ "name": "Atuatus",
+ "pos_x": -143.53125,
+ "pos_y": -32.3125,
+ "pos_z": 36.5,
+ "stations": "Baudin Relay,Popov Freeport"
+ },
+ {
+ "name": "Atum",
+ "pos_x": 49.96875,
+ "pos_y": 17.625,
+ "pos_z": 5.59375,
+ "stations": "Bottego Hub,Leckie City"
+ },
+ {
+ "name": "Atun",
+ "pos_x": 75.4375,
+ "pos_y": -24.9375,
+ "pos_z": -132,
+ "stations": "Hasse's Claim,Sargent Prospect"
+ },
+ {
+ "name": "Atung Mussa",
+ "pos_x": 106.5,
+ "pos_y": -53.25,
+ "pos_z": 122.125,
+ "stations": "Cortes Depot,Gaspar de Portola's Exile,Stafford Installation"
+ },
+ {
+ "name": "Atuntha",
+ "pos_x": -26.1875,
+ "pos_y": -97.8125,
+ "pos_z": 105,
+ "stations": "Hooker Holdings,Nojiri City,von Biela Port,Green Arsenal,Anderson Terminal"
+ },
+ {
+ "name": "Atunung",
+ "pos_x": 150.75,
+ "pos_y": -207.34375,
+ "pos_z": 5.4375,
+ "stations": "Ryan Stop"
+ },
+ {
+ "name": "Auaker",
+ "pos_x": 125.1875,
+ "pos_y": -134.75,
+ "pos_z": -90.96875,
+ "stations": "Peebles Mines"
+ },
+ {
+ "name": "Auakereks",
+ "pos_x": -122.9375,
+ "pos_y": -42.03125,
+ "pos_z": -26.0625,
+ "stations": "Morin Dock,Otto Port,Seddon Port,Chalker Market"
+ },
+ {
+ "name": "Audheim",
+ "pos_x": -88,
+ "pos_y": -109.09375,
+ "pos_z": -49.78125,
+ "stations": "Lanier Ring,Cadamosto Terminal,Bradbury Hub,Nordenskiold Hub"
+ },
+ {
+ "name": "Audhenu",
+ "pos_x": 41.125,
+ "pos_y": -181.59375,
+ "pos_z": -59.46875,
+ "stations": "Ikeya Station,Burnham Prospect"
+ },
+ {
+ "name": "Audhr",
+ "pos_x": 7.15625,
+ "pos_y": -57.8125,
+ "pos_z": 57.4375,
+ "stations": "Blackwell Port,Godwin Ring,Somerset Depot,McArthur Station"
+ },
+ {
+ "name": "Audhuma",
+ "pos_x": 25.34375,
+ "pos_y": 72.4375,
+ "pos_z": 90.25,
+ "stations": "Wul's Folly,Rothman Vision,Kaku Terminal,Al-Farabi Dock"
+ },
+ {
+ "name": "Audhumbwe",
+ "pos_x": -60.40625,
+ "pos_y": -20.34375,
+ "pos_z": 98.75,
+ "stations": "Cartwright Refinery,Tani Works"
+ },
+ {
+ "name": "Audhumis",
+ "pos_x": -111.34375,
+ "pos_y": 32.0625,
+ "pos_z": -102.71875,
+ "stations": "Hedin Dock"
+ },
+ {
+ "name": "Audhumla",
+ "pos_x": 28.375,
+ "pos_y": -82.375,
+ "pos_z": -24.21875,
+ "stations": "Filipchenko Gateway,Galvani Hub,Noli Settlement,Reamy Beacon,Virchow City"
+ },
+ {
+ "name": "Audumasi",
+ "pos_x": 15.15625,
+ "pos_y": 37.09375,
+ "pos_z": -158.0625,
+ "stations": "Janes Station,Garcia Bastion,Lander Observatory"
+ },
+ {
+ "name": "Audumui",
+ "pos_x": -33.5625,
+ "pos_y": -65.21875,
+ "pos_z": 102.59375,
+ "stations": "Wnuk-Lipinski Relay,Skolem Point,Smith Dock"
+ },
+ {
+ "name": "Audusi",
+ "pos_x": -115.71875,
+ "pos_y": 16.53125,
+ "pos_z": -21.09375,
+ "stations": "Woolley City,Dall Terminal,Dickson Works,Watts Observatory"
+ },
+ {
+ "name": "Audusiani",
+ "pos_x": -128.8125,
+ "pos_y": 44.625,
+ "pos_z": 63.3125,
+ "stations": "Nicolet Dock,Williams Gateway"
+ },
+ {
+ "name": "Aulebegus",
+ "pos_x": -28.15625,
+ "pos_y": -44.21875,
+ "pos_z": -47.09375,
+ "stations": "Redi Vision"
+ },
+ {
+ "name": "Aulendiae",
+ "pos_x": -108.8125,
+ "pos_y": -45.625,
+ "pos_z": 104.5625,
+ "stations": "Drew Colony,Springer Depot,Aleksandrov Colony"
+ },
+ {
+ "name": "Aulerci",
+ "pos_x": -1.09375,
+ "pos_y": -92.0625,
+ "pos_z": 120.09375,
+ "stations": "Tanner Vision,Van Scyoc Vision,Rose Dock,Hume's Progress"
+ },
+ {
+ "name": "Aulin",
+ "pos_x": -19.6875,
+ "pos_y": 32.6875,
+ "pos_z": 4.75,
+ "stations": "Aulin Enterprise,Kuo City,Edwards Ring,Simmons' Progress"
+ },
+ {
+ "name": "Aulis",
+ "pos_x": -16.46875,
+ "pos_y": 44.1875,
+ "pos_z": -11.4375,
+ "stations": "Dezhurov Gateway,Fossey Station,Hirasawa Orbital,Fancher's Pride,Schmitz's Progress,Xing Hub"
+ },
+ {
+ "name": "Aunochis",
+ "pos_x": 58.8125,
+ "pos_y": 14.03125,
+ "pos_z": -45.71875,
+ "stations": "Ray Relay,Fuglesang Base"
+ },
+ {
+ "name": "Aura",
+ "pos_x": -16.625,
+ "pos_y": 32.15625,
+ "pos_z": 55.5625,
+ "stations": "Berezovoy Orbital,Hopper City,Dobrovolskiy Hub,Atkov Ring,Hurley Dock,Aikin Enterprise,Chomsky Prospect,Thuot Gateway,Lupoff Bastion,Burbank Horizons"
+ },
+ {
+ "name": "Auradjan",
+ "pos_x": -124.28125,
+ "pos_y": -12.21875,
+ "pos_z": 110.125,
+ "stations": "Malzberg Station,Tapinas City,Daley Reach"
+ },
+ {
+ "name": "Aurai",
+ "pos_x": 0.9375,
+ "pos_y": -47.8125,
+ "pos_z": 46.28125,
+ "stations": "Hill Camp,Adams Prospect,Heisenberg Installation"
+ },
+ {
+ "name": "Aurawala",
+ "pos_x": 146.09375,
+ "pos_y": -104.75,
+ "pos_z": 88.71875,
+ "stations": "Dilworth Stop"
+ },
+ {
+ "name": "Aurea",
+ "pos_x": -88,
+ "pos_y": 23.53125,
+ "pos_z": -8.28125,
+ "stations": "Powell Outpost,Norman Silo,Waldrop Plant"
+ },
+ {
+ "name": "Aurgel",
+ "pos_x": 89.125,
+ "pos_y": -96.28125,
+ "pos_z": 32.4375,
+ "stations": "Slade Settlement"
+ },
+ {
+ "name": "Aurgens",
+ "pos_x": 53.46875,
+ "pos_y": -198.90625,
+ "pos_z": -21.0625,
+ "stations": "Sitter Vision,Clairaut Point"
+ },
+ {
+ "name": "Aurora",
+ "pos_x": 53.34375,
+ "pos_y": 41.78125,
+ "pos_z": -61.46875,
+ "stations": "Collins Gateway,Crown Keep,Walter Gateway,Phillifent Landing,Hauck Gateway,Cooper Orbital,Anderson Hub"
+ },
+ {
+ "name": "Aurora Astrum",
+ "pos_x": -9533.25,
+ "pos_y": -888.9375,
+ "pos_z": 19796.90625,
+ "stations": "Prisma Renata"
+ },
+ {
+ "name": "Aurui",
+ "pos_x": 100.3125,
+ "pos_y": -47.9375,
+ "pos_z": 95.65625,
+ "stations": "Roth Dock,Littrow Prospect,Laurent Keep"
+ },
+ {
+ "name": "Aurus",
+ "pos_x": -139.84375,
+ "pos_y": -81.34375,
+ "pos_z": -12.6875,
+ "stations": "Ericsson Settlement,Guidoni Orbital,Payne Reach"
+ },
+ {
+ "name": "Aurvashi",
+ "pos_x": 110.65625,
+ "pos_y": -173.75,
+ "pos_z": 125.53125,
+ "stations": "Cheranovsky Port,Shoemaker Base,al-Din al-Urdi Terminal,Vaisala Enterprise"
+ },
+ {
+ "name": "Aurvasude",
+ "pos_x": 143.0625,
+ "pos_y": -211.3125,
+ "pos_z": 23.78125,
+ "stations": "Schiaparelli Depot"
+ },
+ {
+ "name": "Ausan",
+ "pos_x": -49.5,
+ "pos_y": -12.09375,
+ "pos_z": -69.40625,
+ "stations": "Ziemkiewicz Settlement,Bear Settlement"
+ },
+ {
+ "name": "Ausantuatel",
+ "pos_x": 128.875,
+ "pos_y": -54.8125,
+ "pos_z": -9.59375,
+ "stations": "Shoemaker Orbital,Cummings Hub,Tiedemann Point"
+ },
+ {
+ "name": "Auscabs",
+ "pos_x": 56.1875,
+ "pos_y": 117.21875,
+ "pos_z": 59.59375,
+ "stations": "Fulton Hangar,Virtanen Dock"
+ },
+ {
+ "name": "Auscani",
+ "pos_x": 157.3125,
+ "pos_y": -52.46875,
+ "pos_z": 51.125,
+ "stations": "Smith Colony,Veblen Barracks"
+ },
+ {
+ "name": "Auscapaka",
+ "pos_x": 57.28125,
+ "pos_y": 119.1875,
+ "pos_z": -84.78125,
+ "stations": "Frobenius Enterprise,Forfait Base,Fast Vision"
+ },
+ {
+ "name": "Auschauts",
+ "pos_x": 135.90625,
+ "pos_y": -81.96875,
+ "pos_z": 39.09375,
+ "stations": "Flynn Hub,Clebsch Refinery,Noon Vista"
+ },
+ {
+ "name": "Ausci",
+ "pos_x": -120.59375,
+ "pos_y": -37.09375,
+ "pos_z": -94.59375,
+ "stations": "Iqbal's Folly,Saberhagen Reach,Vlamingh Keep"
+ },
+ {
+ "name": "Auseriabiku",
+ "pos_x": -80.03125,
+ "pos_y": -86.625,
+ "pos_z": 144.84375,
+ "stations": "Steinmuller Horizons,Brooks Terminal,Malcolm Depot"
+ },
+ {
+ "name": "Auserid",
+ "pos_x": 147.78125,
+ "pos_y": -215.8125,
+ "pos_z": 85.15625,
+ "stations": "Abe Landing,Faber Mines,Andrews Terminal"
+ },
+ {
+ "name": "Auserine",
+ "pos_x": 23.75,
+ "pos_y": -22.21875,
+ "pos_z": 64.65625,
+ "stations": "Norton Holdings,Runco Prospect"
+ },
+ {
+ "name": "Auserktomis",
+ "pos_x": -71.09375,
+ "pos_y": 44.90625,
+ "pos_z": 155.8125,
+ "stations": "Gregory Prospect,Larbalestier's Progress"
+ },
+ {
+ "name": "Ausermoduro",
+ "pos_x": -0.53125,
+ "pos_y": -153.9375,
+ "pos_z": -24.65625,
+ "stations": "Trumpler Mines"
+ },
+ {
+ "name": "Auso",
+ "pos_x": 164.25,
+ "pos_y": -194.90625,
+ "pos_z": 85.53125,
+ "stations": "Vess Station,Thorne City"
+ },
+ {
+ "name": "Ausrintini",
+ "pos_x": 104.59375,
+ "pos_y": -86.59375,
+ "pos_z": -29.8125,
+ "stations": "Maanen Outpost"
+ },
+ {
+ "name": "Auss",
+ "pos_x": 23.15625,
+ "pos_y": -220.40625,
+ "pos_z": 14.75,
+ "stations": "Flammarion Outpost"
+ },
+ {
+ "name": "Austa",
+ "pos_x": 138.59375,
+ "pos_y": -100.15625,
+ "pos_z": -19.59375,
+ "stations": "Slipher Orbital,Ross' Claim,Dassault Installation,Derekas Vision,Andoyer Depot"
+ },
+ {
+ "name": "Auste",
+ "pos_x": 119.71875,
+ "pos_y": -59.78125,
+ "pos_z": -80.09375,
+ "stations": "Perry Terminal,Moriarty Landing,Martin Silo,Arnarson City"
+ },
+ {
+ "name": "Austeja",
+ "pos_x": 54.15625,
+ "pos_y": -15.0625,
+ "pos_z": -133.84375,
+ "stations": "Lagrange Platform"
+ },
+ {
+ "name": "Austeret",
+ "pos_x": -34.34375,
+ "pos_y": 101.375,
+ "pos_z": -94.53125,
+ "stations": "Beatty Settlement,Dorsey Dock,Otto Depot"
+ },
+ {
+ "name": "Auta Isuang",
+ "pos_x": -121.28125,
+ "pos_y": -98.84375,
+ "pos_z": 20.78125,
+ "stations": "Evans Settlement,Treshchov Terminal,Dunbar Orbital,Mendez Point"
+ },
+ {
+ "name": "Autahenetsi",
+ "pos_x": 8.59375,
+ "pos_y": -70,
+ "pos_z": -29.875,
+ "stations": "Artyukhin Ring,Aldiss' Folly,Boe Enterprise,Freud City"
+ },
+ {
+ "name": "Autamkindia",
+ "pos_x": -1.0625,
+ "pos_y": -163.75,
+ "pos_z": 60.5625,
+ "stations": "Gutierrez Arsenal,Knorre Colony,Sikorsky Station"
+ },
+ {
+ "name": "Auteini",
+ "pos_x": 139.25,
+ "pos_y": -135.78125,
+ "pos_z": 61.25,
+ "stations": "Pickering Mine"
+ },
+ {
+ "name": "Autians",
+ "pos_x": -30.9375,
+ "pos_y": -130.03125,
+ "pos_z": 78.53125,
+ "stations": "Bailly Stop"
+ },
+ {
+ "name": "Autinestes",
+ "pos_x": 140.84375,
+ "pos_y": -179.59375,
+ "pos_z": -49.4375,
+ "stations": "McKee Works,Saarinen Mines,Grushin Station,Malchiodi Depot"
+ },
+ {
+ "name": "Autribeb",
+ "pos_x": -18.71875,
+ "pos_y": -32.75,
+ "pos_z": 111.90625,
+ "stations": "Malpighi Station"
+ },
+ {
+ "name": "Autrimnit",
+ "pos_x": -193.96875,
+ "pos_y": 47.78125,
+ "pos_z": 10.59375,
+ "stations": "Minkowski Relay,Caryanda Dock,Kratman Enterprise,Auer's Folly"
+ },
+ {
+ "name": "Autrimpe",
+ "pos_x": 56.125,
+ "pos_y": -153.59375,
+ "pos_z": -22.1875,
+ "stations": "Wallis Hub,Leberecht Tempel Vision,Luther Colony,Brahmagupta Terminal"
+ },
+ {
+ "name": "Autroch",
+ "pos_x": 31.125,
+ "pos_y": -139.96875,
+ "pos_z": 157.6875,
+ "stations": "Cayley Horizons,Okuni Port,Takahashi Vision,Johri Settlement"
+ },
+ {
+ "name": "Avalo",
+ "pos_x": 178.78125,
+ "pos_y": -132.75,
+ "pos_z": 61.34375,
+ "stations": "MacCready Orbital,Nomen Landing,Naubakht Prospect,Robert Platform"
+ },
+ {
+ "name": "Avalon",
+ "pos_x": 50.1875,
+ "pos_y": -132.96875,
+ "pos_z": 36.1875,
+ "stations": "Akiyama Vision,Eilenberg Landing,Noctilux,Persephone,Houten-Groeneveld Relay"
+ },
+ {
+ "name": "Avan",
+ "pos_x": -77.3125,
+ "pos_y": -17.78125,
+ "pos_z": 92.0625,
+ "stations": "Timofeyevich Co-operative,Nobel Dock,Crown Beacon"
+ },
+ {
+ "name": "Avandhs",
+ "pos_x": 151.59375,
+ "pos_y": 49.53125,
+ "pos_z": 20.875,
+ "stations": "Ore Point"
+ },
+ {
+ "name": "Avane",
+ "pos_x": 28.25,
+ "pos_y": 29.4375,
+ "pos_z": 146,
+ "stations": "Darnielle Plant,Fowler Landing,Thurston Bastion,Panshin Prospect"
+ },
+ {
+ "name": "Avanty",
+ "pos_x": 59.34375,
+ "pos_y": 143.8125,
+ "pos_z": 9.8125,
+ "stations": "Huberath Enterprise,Phillips Mines"
+ },
+ {
+ "name": "Avarigines",
+ "pos_x": 67.8125,
+ "pos_y": 59.53125,
+ "pos_z": -15.25,
+ "stations": "Ross Survey"
+ },
+ {
+ "name": "Avata",
+ "pos_x": 56.78125,
+ "pos_y": -169.34375,
+ "pos_z": 169.4375,
+ "stations": "Allen St. John Orbital,Cummings Relay"
+ },
+ {
+ "name": "Avataultam",
+ "pos_x": -150.15625,
+ "pos_y": -3.78125,
+ "pos_z": 27.03125,
+ "stations": "Fraley Hub,Good Enterprise"
+ },
+ {
+ "name": "Avaticus",
+ "pos_x": -143.34375,
+ "pos_y": 10.375,
+ "pos_z": 104.8125,
+ "stations": "Bosch Colony,Liwei Settlement,Sturgeon Landing"
+ },
+ {
+ "name": "Avik",
+ "pos_x": 13.96875,
+ "pos_y": -4.59375,
+ "pos_z": -6,
+ "stations": "Bruce Prospect"
+ },
+ {
+ "name": "Avikarli",
+ "pos_x": -4.0625,
+ "pos_y": 140.03125,
+ "pos_z": 35.78125,
+ "stations": "Normand Hub,Killough Vision,Stith Terminal,Scheerbart Installation,Wylie Barracks,Bacigalupi Base"
+ },
+ {
+ "name": "Awa",
+ "pos_x": 83.6875,
+ "pos_y": -22,
+ "pos_z": 131.46875,
+ "stations": "Jacquard Vision,Noakes Orbital,Norman Survey"
+ },
+ {
+ "name": "Awabakal",
+ "pos_x": 19.28125,
+ "pos_y": -236.21875,
+ "pos_z": 81.21875,
+ "stations": "Mobius Horizons,Elst Port"
+ },
+ {
+ "name": "Awabara",
+ "pos_x": -131.125,
+ "pos_y": -11.21875,
+ "pos_z": 17.625,
+ "stations": "Laveykin Mines,Rothman Hub,Vries Horizons,Leslie Colony"
+ },
+ {
+ "name": "Awabatamori",
+ "pos_x": -110.78125,
+ "pos_y": 39.46875,
+ "pos_z": -35.59375,
+ "stations": "Caryanda Freeport,Carver Installation,Stiegler Colony,Napier Prospect,Samos Point"
+ },
+ {
+ "name": "Awang",
+ "pos_x": 82.875,
+ "pos_y": -19.40625,
+ "pos_z": 94.40625,
+ "stations": "Clark Holdings,Bernoulli Base"
+ },
+ {
+ "name": "Awanti",
+ "pos_x": -114.03125,
+ "pos_y": 23.375,
+ "pos_z": -99.53125,
+ "stations": "Solovyov Mine"
+ },
+ {
+ "name": "Awara",
+ "pos_x": 67.21875,
+ "pos_y": -72.65625,
+ "pos_z": -127.34375,
+ "stations": "Haisheng Colony,Jendrassik Port,Manarov Landing,Malaspina Refinery,Usachov Works"
+ },
+ {
+ "name": "Awarilo",
+ "pos_x": -30.8125,
+ "pos_y": 105.4375,
+ "pos_z": 95.34375,
+ "stations": "Steiner Dock,Jacobi Horizons"
+ },
+ {
+ "name": "Awawa",
+ "pos_x": -0.0625,
+ "pos_y": 66.0625,
+ "pos_z": 42.21875,
+ "stations": "Drake Settlement,Darboux Orbital"
+ },
+ {
+ "name": "Awawad",
+ "pos_x": -101.75,
+ "pos_y": 37.8125,
+ "pos_z": -10.375,
+ "stations": "Stein Installation,Bosch Hangar,Bardeen City,Dukaj Prospect"
+ },
+ {
+ "name": "Awawadja",
+ "pos_x": 79.96875,
+ "pos_y": -167.84375,
+ "pos_z": 154.5,
+ "stations": "Kanai Depot"
+ },
+ {
+ "name": "Awawar",
+ "pos_x": -54.75,
+ "pos_y": 76.53125,
+ "pos_z": 39.1875,
+ "stations": "Cartwright Orbital,Nye Orbital,Avdeyev Station,VanderMeer Hub"
+ },
+ {
+ "name": "Aweti",
+ "pos_x": 48.34375,
+ "pos_y": -67.90625,
+ "pos_z": -126.09375,
+ "stations": "Yolen Oasis,Shapiro Settlement"
+ },
+ {
+ "name": "Awindji",
+ "pos_x": -117.5,
+ "pos_y": -18.34375,
+ "pos_z": 41.9375,
+ "stations": "Kononenko Vision"
+ },
+ {
+ "name": "Awindjibata",
+ "pos_x": -16.28125,
+ "pos_y": -25.65625,
+ "pos_z": 15.59375,
+ "stations": "Karl Diesel Hub"
+ },
+ {
+ "name": "Awithamu",
+ "pos_x": -170.625,
+ "pos_y": -52.65625,
+ "pos_z": -2.5625,
+ "stations": "Cardano Orbital,Mitra Settlement"
+ },
+ {
+ "name": "Awngtei",
+ "pos_x": -133.5625,
+ "pos_y": 10.21875,
+ "pos_z": -58.5625,
+ "stations": "Coleman Beacon,Wankel Dock,Thomas Barracks"
+ },
+ {
+ "name": "Awngtei Ji",
+ "pos_x": -41.78125,
+ "pos_y": -144.15625,
+ "pos_z": 6.78125,
+ "stations": "Fallows Colony"
+ },
+ {
+ "name": "Awngthim",
+ "pos_x": -133.40625,
+ "pos_y": -9.84375,
+ "pos_z": 30.40625,
+ "stations": "Gaffney Port,Mitchell Landing,Collins Enterprise,Robinson Dock,Heaviside Point,Slade Prospect"
+ },
+ {
+ "name": "Awonai",
+ "pos_x": -2.46875,
+ "pos_y": 41.3125,
+ "pos_z": -96.25,
+ "stations": "Boming Port,Knapp Station,Nusslein-Volhard Hub,Ohm Hub,White Market,Quimby Port,Henry Port,Gohar Orbital,Desargues Gateway,Crown Prospect"
+ },
+ {
+ "name": "Awonawilona",
+ "pos_x": 118.78125,
+ "pos_y": -102.34375,
+ "pos_z": 90.375,
+ "stations": "Minkowski Works"
+ },
+ {
+ "name": "Awongbal",
+ "pos_x": -102.28125,
+ "pos_y": 34.0625,
+ "pos_z": -121.8125,
+ "stations": "Lupoff Ring,Cortes Port,Russell Terminal,Chios Relay,al-Haytham Bastion"
+ },
+ {
+ "name": "Awonggalis",
+ "pos_x": -3.28125,
+ "pos_y": -63.6875,
+ "pos_z": 145.8125,
+ "stations": "Schumacher Vision,Pribylov Depot,Thiele Orbital"
+ },
+ {
+ "name": "AY Indi",
+ "pos_x": 26.9375,
+ "pos_y": -33.59375,
+ "pos_z": 31.28125,
+ "stations": "Farkas Dock,Soper Hub"
+ },
+ {
+ "name": "Ayethi",
+ "pos_x": 29.28125,
+ "pos_y": -71.09375,
+ "pos_z": -21.71875,
+ "stations": "Brislingholm,Muninn's Nest,Pacheco City,Fox Horizons,Palitzsch Installation"
+ },
+ {
+ "name": "Aymarahuara",
+ "pos_x": -151.5,
+ "pos_y": 39.40625,
+ "pos_z": -74.1875,
+ "stations": "Marconi's Claim,Zhen Lab,Chilton Landing"
+ },
+ {
+ "name": "Aymariyot",
+ "pos_x": 23.1875,
+ "pos_y": 78.25,
+ "pos_z": 63.34375,
+ "stations": "Weitz Station,Broglie Gateway,Tombaugh Gateway,Lazutkin Enterprise,Koch Enterprise,Roberts Hub,Swanson Vision"
+ },
+ {
+ "name": "Aymiay",
+ "pos_x": -53.5,
+ "pos_y": -28.8125,
+ "pos_z": -63.09375,
+ "stations": "Lloydport,Coblentz Relay,Fort Diamond,Grimwood Vision,Armstrong Installation"
+ },
+ {
+ "name": "Aymifa",
+ "pos_x": 66.5,
+ "pos_y": -109.25,
+ "pos_z": 1.875,
+ "stations": "Lomonosov Colony,Polya Coliseum,Tsunenaga Enterprise,Coggia Port,Hunziker Orbital,Wallis Arsenal,Lee Settlement"
+ },
+ {
+ "name": "Ayora",
+ "pos_x": -84.75,
+ "pos_y": -75.1875,
+ "pos_z": 70.3125,
+ "stations": "Kidman Outpost,Cartwright Hub"
+ },
+ {
+ "name": "Ayorente",
+ "pos_x": -74.5,
+ "pos_y": 29.625,
+ "pos_z": 130.1875,
+ "stations": "Serling Reach,Hunt Platform"
+ },
+ {
+ "name": "Ayores",
+ "pos_x": -1.625,
+ "pos_y": -28.65625,
+ "pos_z": 140.5625,
+ "stations": "Cook Ring,al-Khayyam's Folly,Salgari Relay"
+ },
+ {
+ "name": "Ayoresi",
+ "pos_x": -70.1875,
+ "pos_y": -14.125,
+ "pos_z": 81.5625,
+ "stations": "Wilson City,Antonio de Andrade Refinery"
+ },
+ {
+ "name": "Ayoro",
+ "pos_x": -41.46875,
+ "pos_y": -12.1875,
+ "pos_z": 99.78125,
+ "stations": "Oliver Dock"
+ },
+ {
+ "name": "Ayoro Nage",
+ "pos_x": -34.5625,
+ "pos_y": -110.125,
+ "pos_z": -23.875,
+ "stations": "Ashby Terminal,Bloomfield Gateway,Ham Dock,Coke Legacy"
+ },
+ {
+ "name": "Ayorubang",
+ "pos_x": 117.5625,
+ "pos_y": -132.375,
+ "pos_z": 155.25,
+ "stations": "Naburimannu Hub,Hoffmeister Station,Vasilyev's Folly"
+ },
+ {
+ "name": "Ayorun",
+ "pos_x": 90.34375,
+ "pos_y": -131.5625,
+ "pos_z": -86.375,
+ "stations": "Rubin Terminal,Arend Station,Yerka Dock"
+ },
+ {
+ "name": "Ayu",
+ "pos_x": 148.6875,
+ "pos_y": -85.59375,
+ "pos_z": 27.96875,
+ "stations": "Winthrop Mines,Trimble Hub,Roe Observatory"
+ },
+ {
+ "name": "AZ Cancri",
+ "pos_x": 19.65625,
+ "pos_y": 26.84375,
+ "pos_z": -38.09375,
+ "stations": "Prunariu Laboratory,Fisher Station,Valdes Prospect,Mitchell Park,Galton Base"
+ },
+ {
+ "name": "Azaka",
+ "pos_x": -43.28125,
+ "pos_y": -34.6875,
+ "pos_z": 33.21875,
+ "stations": "Bresnik Orbital,Carrier Station,Cheli Bastion"
+ },
+ {
+ "name": "Azaladshu",
+ "pos_x": 60.9375,
+ "pos_y": 10.34375,
+ "pos_z": -1.09375,
+ "stations": "Eratosthenes Ring,Buffett Vision,Junlong Terminal,Acaba Terminal,Aksyonov Hub,Battuta Palace,Linenger Dock,Boming Terminal"
+ },
+ {
+ "name": "Azalai",
+ "pos_x": -154.53125,
+ "pos_y": 21.1875,
+ "pos_z": -11.1875,
+ "stations": "Oefelein Hub,Pelt Depot"
+ },
+ {
+ "name": "Azaleach",
+ "pos_x": -81.28125,
+ "pos_y": 60.78125,
+ "pos_z": -121.46875,
+ "stations": "Leoniceno Orbital"
+ },
+ {
+ "name": "Azaliba",
+ "pos_x": 20.90625,
+ "pos_y": -26.125,
+ "pos_z": 131.53125,
+ "stations": "Burgess Ring"
+ },
+ {
+ "name": "Azalis",
+ "pos_x": -38.28125,
+ "pos_y": 26.875,
+ "pos_z": 119.3125,
+ "stations": "Fontana Survey,Asaro Vision,Behring Outpost"
+ },
+ {
+ "name": "Azha",
+ "pos_x": 10.25,
+ "pos_y": -111.4375,
+ "pos_z": -78.21875,
+ "stations": "Locke Gateway,Berliner Enterprise"
+ },
+ {
+ "name": "Azrael",
+ "pos_x": 85.71875,
+ "pos_y": -104.5625,
+ "pos_z": 16.25,
+ "stations": "Rafferty's Mobius,Extra Refinery,Searle Port,Guth Dock,Friedman Base"
+ },
+ {
+ "name": "Azraia",
+ "pos_x": 25.90625,
+ "pos_y": -167,
+ "pos_z": 69.59375,
+ "stations": "van Rhijn Dock,Merril Hub"
+ },
+ {
+ "name": "Aztlan",
+ "pos_x": 80.8125,
+ "pos_y": -133.71875,
+ "pos_z": 6.40625,
+ "stations": "Tannhauser Gate"
+ },
+ {
+ "name": "b Herculis",
+ "pos_x": -39.78125,
+ "pos_y": 19.03125,
+ "pos_z": 25.6875,
+ "stations": "Thuot Horizons,Schwann Settlement,Ray Horizons"
+ },
+ {
+ "name": "B'Titus",
+ "pos_x": -28.6875,
+ "pos_y": 59.4375,
+ "pos_z": -81.90625,
+ "stations": "al-Khowarizmi Settlement,Harris-Johnson"
+ },
+ {
+ "name": "b2 Carinae",
+ "pos_x": 84.5625,
+ "pos_y": -12.875,
+ "pos_z": 10.4375,
+ "stations": "Williams Oasis,Asire Enterprise,Yourkevitch Enterprise,Hewish Port,Weinbaum Prospect,Dixon Ring,Kawasato City,Secchi Hub,Bergerac Point,Mechain Vision,Herbert Holdings,Cummings Oasis,Muller Orbital,Herschel City"
+ },
+ {
+ "name": "b3 Hydrae",
+ "pos_x": 82.4375,
+ "pos_y": 57.40625,
+ "pos_z": -0.78125,
+ "stations": "Song Orbital"
+ },
+ {
+ "name": "Ba Babal",
+ "pos_x": 20.1875,
+ "pos_y": 114.28125,
+ "pos_z": 115.625,
+ "stations": "Caryanda Orbital,Amundsen Ring,Mitra Port,Andree Holdings,Krusvar Depot"
+ },
+ {
+ "name": "Ba Bao",
+ "pos_x": -62.75,
+ "pos_y": 75.53125,
+ "pos_z": -42.3125,
+ "stations": "Fuca Market,Aguirre Orbital"
+ },
+ {
+ "name": "Ba Bhuti",
+ "pos_x": -90.9375,
+ "pos_y": 63.0625,
+ "pos_z": 17.125,
+ "stations": "Lockhart Station,Whitney Station"
+ },
+ {
+ "name": "Ba Devaci",
+ "pos_x": -128.875,
+ "pos_y": 57.15625,
+ "pos_z": -49.84375,
+ "stations": "Bardeen Hub,Still Enterprise"
+ },
+ {
+ "name": "Ba Dhor",
+ "pos_x": 55.5,
+ "pos_y": -177.75,
+ "pos_z": -4.5625,
+ "stations": "Schumacher Hub,Sei Station"
+ },
+ {
+ "name": "Ba Jonandja",
+ "pos_x": -18.53125,
+ "pos_y": 36.09375,
+ "pos_z": -91.78125,
+ "stations": "Akers Ring,Bursch City,Acton Dock,Wylie's Progress,Kingsmill Landing"
+ },
+ {
+ "name": "Ba Narfi",
+ "pos_x": 173.03125,
+ "pos_y": -140.5,
+ "pos_z": -10.9375,
+ "stations": "Kemurdzhian Station,Kobayashi Vision,Freycinet Silo"
+ },
+ {
+ "name": "Ba Nari",
+ "pos_x": 46.1875,
+ "pos_y": -126.46875,
+ "pos_z": 1.9375,
+ "stations": "de Seversky Plant,Gottlob Frege Base,Hereford Keep"
+ },
+ {
+ "name": "Ba Narr",
+ "pos_x": -95.65625,
+ "pos_y": -100.3125,
+ "pos_z": -25.34375,
+ "stations": "Kessel Port,Cummings Terminal,Szebehely Terminal,Hoyle City"
+ },
+ {
+ "name": "Ba Nefes",
+ "pos_x": 64.21875,
+ "pos_y": -91.6875,
+ "pos_z": 160.28125,
+ "stations": "Anderson Hub,Rizvi Dock,Kozin Enterprise"
+ },
+ {
+ "name": "Ba Njok",
+ "pos_x": 107.78125,
+ "pos_y": -94.90625,
+ "pos_z": -123.03125,
+ "stations": "Wolfe Ring"
+ },
+ {
+ "name": "Ba Pin",
+ "pos_x": -163.25,
+ "pos_y": 7.90625,
+ "pos_z": -40.09375,
+ "stations": "Ford Dock,Shunn Dock,Derleth Bastion,Fast Prospect"
+ },
+ {
+ "name": "Ba Po",
+ "pos_x": -18.125,
+ "pos_y": 16.5625,
+ "pos_z": 81.78125,
+ "stations": "Mullane Mines,Korniyenko Settlement,Bella Station,Lewitt Works,Westerfeld Terminal"
+ },
+ {
+ "name": "Ba Pula",
+ "pos_x": 146.21875,
+ "pos_y": -116.40625,
+ "pos_z": 121.0625,
+ "stations": "Cowling Outpost"
+ },
+ {
+ "name": "Ba Xian",
+ "pos_x": -89.6875,
+ "pos_y": -59.5,
+ "pos_z": -9.5,
+ "stations": "Wyndham's Progress,Lasswitz Holdings,Vizcaino Orbital"
+ },
+ {
+ "name": "Ba Xianiya",
+ "pos_x": -104.6875,
+ "pos_y": -101.375,
+ "pos_z": -80.28125,
+ "stations": "Sevastyanov Enterprise,McKay City,Zebrowski Works,Hornby Enterprise,Harrison Horizons"
+ },
+ {
+ "name": "Ba Xinga",
+ "pos_x": 110.15625,
+ "pos_y": 51.90625,
+ "pos_z": -81.4375,
+ "stations": "Gabriel Platform,Hogan Point,Elcano Vision"
+ },
+ {
+ "name": "Baadaga",
+ "pos_x": 138.15625,
+ "pos_y": -3.71875,
+ "pos_z": 40.625,
+ "stations": "Poisson Dock,Aitken Point,Powell Survey"
+ },
+ {
+ "name": "Baal",
+ "pos_x": 83.0625,
+ "pos_y": -140.5,
+ "pos_z": 8.125,
+ "stations": "Stromgren Orbital,Check Orbital,Tousey Orbital,Kippax Settlement,Horrocks Vision,Westphal Hub,Iwamoto Dock,Martin Settlement,Babcock Hub,Oterma Station,Kalam's Progress,Boyajian Legacy,Flammarion Holdings,Behnisch Hub"
+ },
+ {
+ "name": "Babai",
+ "pos_x": 86.3125,
+ "pos_y": -22.9375,
+ "pos_z": -91.21875,
+ "stations": "Cousin Plant,Morris Landing,Isherwood Escape"
+ },
+ {
+ "name": "Babal",
+ "pos_x": 5.8125,
+ "pos_y": -65.53125,
+ "pos_z": 80.96875,
+ "stations": "Sverdrup Terminal,Swanwick Landing,Vian Holdings"
+ },
+ {
+ "name": "Babalung",
+ "pos_x": 119.25,
+ "pos_y": 58.03125,
+ "pos_z": -37.46875,
+ "stations": "Lie Relay,Sauma's Claim,Eisenstein Relay"
+ },
+ {
+ "name": "Babaradhbh",
+ "pos_x": -26.34375,
+ "pos_y": -74.09375,
+ "pos_z": -129.25,
+ "stations": "Yegorov Ring"
+ },
+ {
+ "name": "Babas",
+ "pos_x": 20.46875,
+ "pos_y": 108.4375,
+ "pos_z": -1.46875,
+ "stations": "Binder Orbital,Poisson Terminal,Jones Park"
+ },
+ {
+ "name": "Bacagua",
+ "pos_x": 30.34375,
+ "pos_y": -18.59375,
+ "pos_z": -145.40625,
+ "stations": "Moore Colony,Dedekind Base"
+ },
+ {
+ "name": "Bacamadia",
+ "pos_x": 116.125,
+ "pos_y": 23.09375,
+ "pos_z": 33.625,
+ "stations": "Bethke Station,Krusvar Dock"
+ },
+ {
+ "name": "Bacamu",
+ "pos_x": 153.0625,
+ "pos_y": -106.21875,
+ "pos_z": 24.3125,
+ "stations": "Lloyd Plant,Manning Depot,Tanaka Orbital,Rees Hub"
+ },
+ {
+ "name": "Bacap",
+ "pos_x": 100.90625,
+ "pos_y": -144.1875,
+ "pos_z": 1.9375,
+ "stations": "Morrow Works"
+ },
+ {
+ "name": "Bacara",
+ "pos_x": -28.59375,
+ "pos_y": -81.3125,
+ "pos_z": 40.3125,
+ "stations": "Gerst Dock"
+ },
+ {
+ "name": "Bacarelia",
+ "pos_x": -90.90625,
+ "pos_y": -9.71875,
+ "pos_z": 116.0625,
+ "stations": "Hawley Port,Harrison Base"
+ },
+ {
+ "name": "Bach",
+ "pos_x": 82.875,
+ "pos_y": 3.53125,
+ "pos_z": -147.6875,
+ "stations": "Bulleid Mines"
+ },
+ {
+ "name": "Backluitaji",
+ "pos_x": -44.46875,
+ "pos_y": -152.8125,
+ "pos_z": -60.3125,
+ "stations": "Revin Orbital,Zewail Ring,Krikalev Hub"
+ },
+ {
+ "name": "Backlum",
+ "pos_x": 142.4375,
+ "pos_y": -176.28125,
+ "pos_z": 24.3125,
+ "stations": "Ahnert-Rohlfs Terminal,Resnik Orbital,Kresak Mines,Bischoff Horizons"
+ },
+ {
+ "name": "Backlumba",
+ "pos_x": -163.375,
+ "pos_y": -15.1875,
+ "pos_z": -82.46875,
+ "stations": "Chwedyk Vision,Cook Gateway,Beliaev Gateway,Ostrander's Folly,Peral Terminal"
+ },
+ {
+ "name": "Backlumbha",
+ "pos_x": 108.53125,
+ "pos_y": 52,
+ "pos_z": -61.0625,
+ "stations": "Coulomb Prospect,Hoffman Hub,Chapman Gateway,Bain Dock,Francisco de Almeida Survey"
+ },
+ {
+ "name": "Bactondinks",
+ "pos_x": 82.90625,
+ "pos_y": -85.90625,
+ "pos_z": 11.3125,
+ "stations": "Comper Dock,Maughmer Platform,Cleve Arena,Shajn Terminal"
+ },
+ {
+ "name": "Bactrib",
+ "pos_x": 17.40625,
+ "pos_y": -140.8125,
+ "pos_z": 37.3125,
+ "stations": "Lagrange Terminal,Maunder Terminal,Kojima Vision,Lee Terminal"
+ },
+ {
+ "name": "Bactrimpox",
+ "pos_x": -123.25,
+ "pos_y": 82,
+ "pos_z": -84,
+ "stations": "Coney Enterprise,Tevis Base,Westerfeld Hub,Barreiros Vision,Weber Plant"
+ },
+ {
+ "name": "Badar",
+ "pos_x": 165.65625,
+ "pos_y": -165.5625,
+ "pos_z": 121.6875,
+ "stations": "Farghani Platform,Dyson Terminal,Littlewood Base"
+ },
+ {
+ "name": "Badb",
+ "pos_x": 9.5625,
+ "pos_y": 105.78125,
+ "pos_z": -118.90625,
+ "stations": "Hilbert's Claim,Birmingham Base"
+ },
+ {
+ "name": "Badbadzist",
+ "pos_x": 19.65625,
+ "pos_y": -136.03125,
+ "pos_z": -71.40625,
+ "stations": "Fabian Terminal,Evans Market,Bouvard Ring,Veron Hub"
+ },
+ {
+ "name": "Badbh",
+ "pos_x": 22.46875,
+ "pos_y": -222.75,
+ "pos_z": -43.625,
+ "stations": "Oikawa Stop"
+ },
+ {
+ "name": "Badia",
+ "pos_x": 82.34375,
+ "pos_y": -87.03125,
+ "pos_z": -11.5,
+ "stations": "Kempf Station,Connes Vision,Shaw Terminal,Ostrander Landing"
+ },
+ {
+ "name": "Badimo",
+ "pos_x": 146.625,
+ "pos_y": 81.65625,
+ "pos_z": 18.09375,
+ "stations": "Galois Holdings,Auld Settlement,Coblentz Laboratory,Barth Survey"
+ },
+ {
+ "name": "Badin",
+ "pos_x": 86.03125,
+ "pos_y": 69.25,
+ "pos_z": 116.625,
+ "stations": "Walheim Port,Young's Progress,Haipeng Orbital"
+ },
+ {
+ "name": "Badja",
+ "pos_x": -2.8125,
+ "pos_y": -45,
+ "pos_z": -74.78125,
+ "stations": "Foale Station,Pogue Terminal"
+ },
+ {
+ "name": "Badjalang",
+ "pos_x": 99.46875,
+ "pos_y": -172,
+ "pos_z": 74.40625,
+ "stations": "Adelman Orbital,Coye Colony"
+ },
+ {
+ "name": "Badjarans",
+ "pos_x": -75.21875,
+ "pos_y": -76.96875,
+ "pos_z": 78.8125,
+ "stations": "Riemann Gateway,Zettel Terminal,Boltzmann Hub"
+ },
+ {
+ "name": "Badjarsh",
+ "pos_x": -125.625,
+ "pos_y": 32.96875,
+ "pos_z": 45.5625,
+ "stations": "Dalmas Camp"
+ },
+ {
+ "name": "Badje",
+ "pos_x": -105.875,
+ "pos_y": -120.375,
+ "pos_z": -91.5625,
+ "stations": "Wait Colburn Dock,Sutcliffe Installation,Semeonis Installation,McMahon Terminal"
+ },
+ {
+ "name": "Badjilasta",
+ "pos_x": 100.9375,
+ "pos_y": 11.375,
+ "pos_z": 92.75,
+ "stations": "Al-Farabi Dock,Cenker Terminal"
+ },
+ {
+ "name": "Baedi Di",
+ "pos_x": 128.8125,
+ "pos_y": -98.78125,
+ "pos_z": -14.59375,
+ "stations": "Fowler Relay"
+ },
+ {
+ "name": "Baedu",
+ "pos_x": -125,
+ "pos_y": 62.34375,
+ "pos_z": 90.28125,
+ "stations": "Stephenson Outpost"
+ },
+ {
+ "name": "Baedule",
+ "pos_x": 46.78125,
+ "pos_y": -54.78125,
+ "pos_z": 3.1875,
+ "stations": "Coye Horizons,Shoujing Depot"
+ },
+ {
+ "name": "Bafook",
+ "pos_x": 165.3125,
+ "pos_y": -199.84375,
+ "pos_z": 75.25,
+ "stations": "Knorre Base,Ikeya Prospect,Frimout Landing"
+ },
+ {
+ "name": "BAG 7",
+ "pos_x": -19.03125,
+ "pos_y": 50.90625,
+ "pos_z": 49.125,
+ "stations": "Mullane Terminal,Tsibliyev Gateway,Fisher City,Tull Forum,Cartier's Inheritance"
+ },
+ {
+ "name": "Baga",
+ "pos_x": -110.3125,
+ "pos_y": 15.125,
+ "pos_z": -45.25,
+ "stations": "Stephenson Port,Celebi Prospect,Goeschke Enterprise,Ochoa Port,Lazarev Vision,Binet Enterprise,Willis Hub"
+ },
+ {
+ "name": "Bagai",
+ "pos_x": -63.71875,
+ "pos_y": -36.0625,
+ "pos_z": 69.46875,
+ "stations": "Humphreys' Progress"
+ },
+ {
+ "name": "Bagaita",
+ "pos_x": -53.3125,
+ "pos_y": 118.65625,
+ "pos_z": -75.03125,
+ "stations": "Moskowitz Landing,Bailey Survey"
+ },
+ {
+ "name": "Bagaiteru",
+ "pos_x": -164.34375,
+ "pos_y": -70.21875,
+ "pos_z": -96,
+ "stations": "Snodgrass Freeport,Phillips' Pride"
+ },
+ {
+ "name": "Bagalatuba",
+ "pos_x": 109.6875,
+ "pos_y": 12,
+ "pos_z": 77,
+ "stations": "Stuart Base,Peral Relay,Becquerel Dock"
+ },
+ {
+ "name": "Bagalis",
+ "pos_x": -88.71875,
+ "pos_y": -6.25,
+ "pos_z": -22.90625,
+ "stations": "Stephenson Port,Evans Platform,Borlaug Dock"
+ },
+ {
+ "name": "Bagalya",
+ "pos_x": 173.3125,
+ "pos_y": -98.1875,
+ "pos_z": -69.21875,
+ "stations": "Urkovic Platform,Dyr Port,Morgan Beacon"
+ },
+ {
+ "name": "Bagan",
+ "pos_x": 18.6875,
+ "pos_y": -13.90625,
+ "pos_z": -138.5,
+ "stations": "Lazarev Plant"
+ },
+ {
+ "name": "Baganhau",
+ "pos_x": 22.8125,
+ "pos_y": -166.53125,
+ "pos_z": 92.5,
+ "stations": "Sugano Horizons"
+ },
+ {
+ "name": "Bagaru",
+ "pos_x": 55.46875,
+ "pos_y": -139,
+ "pos_z": 123.75,
+ "stations": "Johri Orbital,Hiyya Colony,McMahon's Folly,Spielberg Prospect"
+ },
+ {
+ "name": "Bagayata",
+ "pos_x": 154.96875,
+ "pos_y": -35.15625,
+ "pos_z": 60.75,
+ "stations": "Laurent Depot,Roth Hub"
+ },
+ {
+ "name": "Bahilo",
+ "pos_x": 131.9375,
+ "pos_y": -223.25,
+ "pos_z": 65.53125,
+ "stations": "De Station,Vasquez de Coronado Terminal,Bayley Base"
+ },
+ {
+ "name": "Bahinga",
+ "pos_x": -46.6875,
+ "pos_y": -142.53125,
+ "pos_z": 67.03125,
+ "stations": "Harawi Ring,Biruni Vision,Bell Terminal,Thurston Relay,Beliaev Terminal"
+ },
+ {
+ "name": "Bahinook",
+ "pos_x": 128.5625,
+ "pos_y": 47.28125,
+ "pos_z": -87,
+ "stations": "Ricci Orbital,Bruce Enterprise,Lorrah Landing,Dupuy de Lome's Folly"
+ },
+ {
+ "name": "Bahpu",
+ "pos_x": 51.6875,
+ "pos_y": -200.4375,
+ "pos_z": 100.1875,
+ "stations": "Thome Holdings,Roberts Enterprise,Scott Camp,Schiaparelli Vision"
+ },
+ {
+ "name": "Baiabi",
+ "pos_x": -77.34375,
+ "pos_y": 19.4375,
+ "pos_z": -145.46875,
+ "stations": "Garneau Port"
+ },
+ {
+ "name": "Baiabozo",
+ "pos_x": -98.5,
+ "pos_y": 50.1875,
+ "pos_z": -89.5625,
+ "stations": "Barratt Orbital,Salam Station,Evans Holdings"
+ },
+ {
+ "name": "Baiamara",
+ "pos_x": 24.4375,
+ "pos_y": 97.0625,
+ "pos_z": 124.25,
+ "stations": "Birkeland Hub"
+ },
+ {
+ "name": "Baiame",
+ "pos_x": -95.96875,
+ "pos_y": 119.1875,
+ "pos_z": -46.6875,
+ "stations": "Shaara Survey,Slusser Survey"
+ },
+ {
+ "name": "Baiawa",
+ "pos_x": 55.4375,
+ "pos_y": -4.78125,
+ "pos_z": 44.5,
+ "stations": "Velho Arsenal,Antonelli Horizons,Dedekind Horizons"
+ },
+ {
+ "name": "Baigag",
+ "pos_x": -52.84375,
+ "pos_y": -22.46875,
+ "pos_z": -106.875,
+ "stations": "Zahn Oasis,Shaw Hub,Schmitt Horizons"
+ },
+ {
+ "name": "Baijio",
+ "pos_x": 61.5625,
+ "pos_y": 17,
+ "pos_z": 61.71875,
+ "stations": "Thomson Station"
+ },
+ {
+ "name": "Baijun",
+ "pos_x": 113.1875,
+ "pos_y": -51.28125,
+ "pos_z": -71.6875,
+ "stations": "Hertz Terminal,Carey Station,Bernoulli's Inheritance,Crippen Terminal,Al-Battani City,Morgan Ring,Harris Gateway"
+ },
+ {
+ "name": "Baijundh",
+ "pos_x": -63.0625,
+ "pos_y": -102.8125,
+ "pos_z": 173.46875,
+ "stations": "Bartoe Beacon,Efremov Beacon,Hutton Beacon"
+ },
+ {
+ "name": "Baijungu",
+ "pos_x": 69.90625,
+ "pos_y": 47.4375,
+ "pos_z": 71.25,
+ "stations": "O'Brien Prospect,Ferguson Market,Gorbatko Enterprise"
+ },
+ {
+ "name": "Baila",
+ "pos_x": 40.65625,
+ "pos_y": 98.59375,
+ "pos_z": -19.96875,
+ "stations": "Celsius Gateway,Weitz Enterprise,Pogue Ring,Ledyard Barracks"
+ },
+ {
+ "name": "Bajang",
+ "pos_x": -157.8125,
+ "pos_y": 25.5625,
+ "pos_z": 76.1875,
+ "stations": "Baraniecki Terminal,Caselberg Enterprise,Mieville Colony"
+ },
+ {
+ "name": "Bajangkhwal",
+ "pos_x": -73.21875,
+ "pos_y": 55.5625,
+ "pos_z": -91.28125,
+ "stations": "Ivanchenkov Prospect"
+ },
+ {
+ "name": "Bajara",
+ "pos_x": 156.8125,
+ "pos_y": -91.5625,
+ "pos_z": 113.125,
+ "stations": "Dunlop Outpost,Ilyushin Survey"
+ },
+ {
+ "name": "Bajau",
+ "pos_x": 6.8125,
+ "pos_y": 91.625,
+ "pos_z": 110.5,
+ "stations": "Clark Horizons,Kirtley Depot"
+ },
+ {
+ "name": "Bajauie",
+ "pos_x": 99.5625,
+ "pos_y": -86.25,
+ "pos_z": -4.53125,
+ "stations": "Apianus Survey,Nomen Landing"
+ },
+ {
+ "name": "Bajie",
+ "pos_x": -116.0625,
+ "pos_y": -23.1875,
+ "pos_z": -127.1875,
+ "stations": "Scortia Gateway,McKay Bastion"
+ },
+ {
+ "name": "Bajito",
+ "pos_x": 81.21875,
+ "pos_y": 151.875,
+ "pos_z": 75.15625,
+ "stations": "Smith Mine,Andree Settlement,Janes Settlement"
+ },
+ {
+ "name": "Bajoji",
+ "pos_x": 92.59375,
+ "pos_y": -32.9375,
+ "pos_z": 95.53125,
+ "stations": "Cramer Gateway,Belyanin Settlement,Beekman Horizons,Gilliland Installation"
+ },
+ {
+ "name": "Bajojiu",
+ "pos_x": 39.21875,
+ "pos_y": -150.65625,
+ "pos_z": -57.375,
+ "stations": "Mizuno City,Bartolomeu de Gusmao City,Nagata Depot"
+ },
+ {
+ "name": "Baka'wa",
+ "pos_x": -86.6875,
+ "pos_y": -32.90625,
+ "pos_z": 5.75,
+ "stations": "Pascal Terminal"
+ },
+ {
+ "name": "Bakabienil",
+ "pos_x": -124.09375,
+ "pos_y": 40.6875,
+ "pos_z": 49.84375,
+ "stations": "Smith Terminal,Pailes Settlement,Bayliss Survey"
+ },
+ {
+ "name": "Bakaduninka",
+ "pos_x": -88.125,
+ "pos_y": -15.1875,
+ "pos_z": 103.5,
+ "stations": "Vinge Ring,al-Khowarizmi Gateway,Turtledove Colony,Ansari Bastion"
+ },
+ {
+ "name": "Bakalaa",
+ "pos_x": 97.4375,
+ "pos_y": 34.375,
+ "pos_z": 97.53125,
+ "stations": "Shaara Platform"
+ },
+ {
+ "name": "Bakalinnama",
+ "pos_x": 2.625,
+ "pos_y": -199.6875,
+ "pos_z": 114.125,
+ "stations": "Narlikar Enterprise,Naboth Hub,Franke Works"
+ },
+ {
+ "name": "Bakara",
+ "pos_x": 109.5,
+ "pos_y": -111.4375,
+ "pos_z": -4.375,
+ "stations": "Arzachel Colony,Arago Camp"
+ },
+ {
+ "name": "Bakas",
+ "pos_x": 84,
+ "pos_y": -2.875,
+ "pos_z": -32.5625,
+ "stations": "Smith Dock,Hoshide Refinery,Murdoch Enterprise"
+ },
+ {
+ "name": "Bakawa",
+ "pos_x": 157.3125,
+ "pos_y": -139.03125,
+ "pos_z": 18.25,
+ "stations": "Hill Tinsley Depot"
+ },
+ {
+ "name": "Baker",
+ "pos_x": 15.59375,
+ "pos_y": -6.28125,
+ "pos_z": 24.5625,
+ "stations": "White Rose,Roentgen Prospect,Wylie Dock"
+ },
+ {
+ "name": "Bakonirtari",
+ "pos_x": -89.21875,
+ "pos_y": -19.59375,
+ "pos_z": 85.59375,
+ "stations": "Gregory Vision"
+ },
+ {
+ "name": "Bakuari",
+ "pos_x": 123.9375,
+ "pos_y": -7.84375,
+ "pos_z": 6.78125,
+ "stations": "Pennington Beacon"
+ },
+ {
+ "name": "Bakulcan",
+ "pos_x": -14.8125,
+ "pos_y": 160.9375,
+ "pos_z": 23.46875,
+ "stations": "Franke Legacy,Obruchev Installation"
+ },
+ {
+ "name": "Bakum",
+ "pos_x": 153.4375,
+ "pos_y": -164.4375,
+ "pos_z": -12.875,
+ "stations": "Lozino-Lozinskiy Mine,MacCurdy Installation"
+ },
+ {
+ "name": "Balaa",
+ "pos_x": -33.65625,
+ "pos_y": -94.71875,
+ "pos_z": 27.34375,
+ "stations": "Ali Resort,Ballard's Inheritance"
+ },
+ {
+ "name": "Balabos",
+ "pos_x": 155.125,
+ "pos_y": 56.5,
+ "pos_z": -1.53125,
+ "stations": "Blalock Terminal"
+ },
+ {
+ "name": "Balaikda",
+ "pos_x": 146.375,
+ "pos_y": -33.15625,
+ "pos_z": 30.46875,
+ "stations": "Chelomey Vision,Potez Station,Spurzem Vision"
+ },
+ {
+ "name": "Balaji",
+ "pos_x": 75.875,
+ "pos_y": 129.28125,
+ "pos_z": 59.46875,
+ "stations": "Bellamy Dock,Nikitin Penal colony,Cousteau Beacon"
+ },
+ {
+ "name": "Balak",
+ "pos_x": -129.375,
+ "pos_y": -73.78125,
+ "pos_z": -20.0625,
+ "stations": "Veach Keep,Land Landing,Runco Outpost"
+ },
+ {
+ "name": "Balakkuru",
+ "pos_x": -18.75,
+ "pos_y": 142.4375,
+ "pos_z": -57.625,
+ "stations": "Plucker Settlement,McMullen Camp"
+ },
+ {
+ "name": "Balampaso",
+ "pos_x": 85.6875,
+ "pos_y": -53.65625,
+ "pos_z": -92.125,
+ "stations": "Banks Platform,Nourse Beacon,Davis Landing"
+ },
+ {
+ "name": "Balams",
+ "pos_x": -34.5625,
+ "pos_y": 12.4375,
+ "pos_z": 129.84375,
+ "stations": "Pennington Dock,Vishweswarayya Holdings,Malpighi City"
+ },
+ {
+ "name": "Balanek",
+ "pos_x": 118.71875,
+ "pos_y": -216.40625,
+ "pos_z": 51.0625,
+ "stations": "Fabricius Point,Olahus Works,Koishikawa Survey"
+ },
+ {
+ "name": "Balangga",
+ "pos_x": -108.625,
+ "pos_y": -82.9375,
+ "pos_z": -33.1875,
+ "stations": "Clebsch's Claim,Coulomb Point,Henricks Port"
+ },
+ {
+ "name": "Balante",
+ "pos_x": -34.21875,
+ "pos_y": -66.1875,
+ "pos_z": -102.15625,
+ "stations": "Laplace Ring"
+ },
+ {
+ "name": "Balanu",
+ "pos_x": 16.15625,
+ "pos_y": -33.84375,
+ "pos_z": 105.8125,
+ "stations": "Remek Dock"
+ },
+ {
+ "name": "Balanukups",
+ "pos_x": 74.65625,
+ "pos_y": -113,
+ "pos_z": 118.71875,
+ "stations": "Lundmark Depot"
+ },
+ {
+ "name": "Balapiru",
+ "pos_x": 7.6875,
+ "pos_y": -162.21875,
+ "pos_z": 123.5,
+ "stations": "Charlois Vision,Rigaux Base"
+ },
+ {
+ "name": "Balardh",
+ "pos_x": -151.28125,
+ "pos_y": 19.8125,
+ "pos_z": -3.5625,
+ "stations": "Alexandria Port"
+ },
+ {
+ "name": "Balastya",
+ "pos_x": -101.34375,
+ "pos_y": 20.75,
+ "pos_z": 118.78125,
+ "stations": "Precourt Terminal,Dalton Enterprise,Ejeta Platform,Rawn Hub,Rowley's Folly"
+ },
+ {
+ "name": "Balatu",
+ "pos_x": 2.65625,
+ "pos_y": -8.3125,
+ "pos_z": 53.65625,
+ "stations": "Haise Dock,Parker Enterprise,Nachtigal Enterprise,Aldiss Installation"
+ },
+ {
+ "name": "Balbus",
+ "pos_x": -27.4375,
+ "pos_y": 44.59375,
+ "pos_z": -75.09375,
+ "stations": "Whymper Settlement,Bao Settlement,Weiss Enterprise"
+ },
+ {
+ "name": "Bald",
+ "pos_x": -67.46875,
+ "pos_y": 33.59375,
+ "pos_z": 145.6875,
+ "stations": "Wagner Installation,Lopez de Haro Installation"
+ },
+ {
+ "name": "Baldeka",
+ "pos_x": -45.8125,
+ "pos_y": 58.875,
+ "pos_z": 56.625,
+ "stations": "Wilmore Enterprise,Pauling Ring,Kopra's Folly"
+ },
+ {
+ "name": "Baldemi",
+ "pos_x": 119.71875,
+ "pos_y": 59.90625,
+ "pos_z": -65.3125,
+ "stations": "Gentle Installation,Zalyotin Landing,Jahn Settlement"
+ },
+ {
+ "name": "Baldr",
+ "pos_x": -43.65625,
+ "pos_y": -61.59375,
+ "pos_z": -68.03125,
+ "stations": "Allen St. John Hub,Keldysh Gateway"
+ },
+ {
+ "name": "Balduk",
+ "pos_x": -27.875,
+ "pos_y": 3.65625,
+ "pos_z": 102.25,
+ "stations": "Dutton Station,Baxter Silo"
+ },
+ {
+ "name": "Baldur",
+ "pos_x": 148.21875,
+ "pos_y": -116.46875,
+ "pos_z": 2,
+ "stations": "Thollon Station,Chelomey Orbital,Wagner Depot,Moriarty Installation"
+ },
+ {
+ "name": "Balie",
+ "pos_x": -86.15625,
+ "pos_y": 120.65625,
+ "pos_z": 58.125,
+ "stations": "Dashiell Dock,Rayhan al-Biruni's Progress,Abel Survey"
+ },
+ {
+ "name": "Balis",
+ "pos_x": 105.6875,
+ "pos_y": -20.96875,
+ "pos_z": 67.78125,
+ "stations": "Hardy Dock,Gorgani Hub,Vyssotsky Works,Bohrmann Orbital,Thornycroft Plant"
+ },
+ {
+ "name": "Baliscii",
+ "pos_x": -43.59375,
+ "pos_y": 37.3125,
+ "pos_z": -122.0625,
+ "stations": "Atwater Depot,Gooch Hangar,Thomas City"
+ },
+ {
+ "name": "Balitcha",
+ "pos_x": 86.875,
+ "pos_y": 65.53125,
+ "pos_z": -50.78125,
+ "stations": "Smeaton Station,Litke Vision,Schwann Keep,Sanger Orbital,Cook Holdings,Richards Settlement,Moseley City,Henry Orbital,Chargaff Dock"
+ },
+ {
+ "name": "Balite",
+ "pos_x": 28.09375,
+ "pos_y": -87.53125,
+ "pos_z": -66.40625,
+ "stations": "Miller Port,Cayley Vista,Egan Station"
+ },
+ {
+ "name": "Balmung",
+ "pos_x": 34.5,
+ "pos_y": -68.59375,
+ "pos_z": 69.375,
+ "stations": "Ruppelt Station,Iben City,Sikorsky Orbital,Mandel Holdings,Gaiman Depot,Chongzhi City,Walotsky Port,Lyne Dock,Lyot Vision,Roe Terminal,Watts Vision,Curtis Hub,Barr Mines,Ryle Orbital,Franklin's Claim"
+ },
+ {
+ "name": "Balmus",
+ "pos_x": -89,
+ "pos_y": 9.375,
+ "pos_z": -52.0625,
+ "stations": "Lockhart Port,vo Dock,Pontes Ring,Brunton Gateway,Lazutkin Orbital,Faris Gateway,Jacquard Terminal,Binnie Enterprise,Brunner Base,Franke Observatory,Morgan Station,Bailey Vision,Jones Depot,Neff Reach"
+ },
+ {
+ "name": "Balo",
+ "pos_x": 152.71875,
+ "pos_y": 51.71875,
+ "pos_z": -38.34375,
+ "stations": "Bottego Dock,Butler Keep,Peano Dock,Cabral Settlement,Farrukh Lab"
+ },
+ {
+ "name": "Balones",
+ "pos_x": -109.15625,
+ "pos_y": -54,
+ "pos_z": -79.96875,
+ "stations": "Tiedemann Dock,Larson Hub,Drake Colony,Brule Landing,Fife Gateway"
+ },
+ {
+ "name": "Baltah'Sine",
+ "pos_x": 85.15625,
+ "pos_y": -56.3125,
+ "pos_z": 40.34375,
+ "stations": "Baltha'Sine Station,Contestabile,Dreyer Survey"
+ },
+ {
+ "name": "Baltalfi",
+ "pos_x": -122.4375,
+ "pos_y": 35.71875,
+ "pos_z": 107.8125,
+ "stations": "Sevastyanov Station,Leibniz City,Samos Market,Savery Gateway"
+ },
+ {
+ "name": "Balte",
+ "pos_x": 150.9375,
+ "pos_y": -139.90625,
+ "pos_z": 98.59375,
+ "stations": "Mattei Outpost"
+ },
+ {
+ "name": "Baltek",
+ "pos_x": -33.59375,
+ "pos_y": 128.1875,
+ "pos_z": -89.90625,
+ "stations": "Akers Works"
+ },
+ {
+ "name": "Balti",
+ "pos_x": 0.25,
+ "pos_y": -76.625,
+ "pos_z": 141.34375,
+ "stations": "Gaensler Landing,Lomonosov Refinery,Macdonald Plant"
+ },
+ {
+ "name": "Balticus",
+ "pos_x": -104.71875,
+ "pos_y": -99.53125,
+ "pos_z": 136.40625,
+ "stations": "Tavernier Vision,Palmer Mines"
+ },
+ {
+ "name": "Bama",
+ "pos_x": -32.21875,
+ "pos_y": -97.875,
+ "pos_z": -0.25,
+ "stations": "Osterbrock Station,Mandel Mines,Dayuan's Progress"
+ },
+ {
+ "name": "Baman",
+ "pos_x": -19.84375,
+ "pos_y": -120.4375,
+ "pos_z": -81.875,
+ "stations": "Zetford City,Karlsefni Beacon,Phillips Refinery"
+ },
+ {
+ "name": "Bamani",
+ "pos_x": -46.40625,
+ "pos_y": -115.1875,
+ "pos_z": -55.40625,
+ "stations": "Hirayama Bastion,Jordan Station,Cleve Orbital,Kelly City,Maclaurin Enterprise,Lukyanenko Enterprise,Anthony de la Roche Station,Nehsi Dock,Miklouho-Maclay's Pride,Shunn Base,Wells Point"
+ },
+ {
+ "name": "Bamarakw",
+ "pos_x": -47.5,
+ "pos_y": -108.1875,
+ "pos_z": -96.1875,
+ "stations": "Nikolayev Port,Darwin Depot,Priestley Dock"
+ },
+ {
+ "name": "Bamazin",
+ "pos_x": -101.40625,
+ "pos_y": -1.84375,
+ "pos_z": 0.40625,
+ "stations": "Aksyonov Mines,Halley Outpost"
+ },
+ {
+ "name": "Bambara",
+ "pos_x": 153.53125,
+ "pos_y": -158.25,
+ "pos_z": 61.03125,
+ "stations": "McManus Depot,Arnold Keep"
+ },
+ {
+ "name": "Bambrigud",
+ "pos_x": 129.46875,
+ "pos_y": 26.0625,
+ "pos_z": -62.84375,
+ "stations": "Volta Terminal,Shirley Relay,Vogel Enterprise,Ewald Base,Anning Ring"
+ },
+ {
+ "name": "Bamileng",
+ "pos_x": -56.34375,
+ "pos_y": 112.78125,
+ "pos_z": 14.8125,
+ "stations": "Russell Landing,Ponce de Leon Station"
+ },
+ {
+ "name": "Bamin",
+ "pos_x": 78.40625,
+ "pos_y": 78.28125,
+ "pos_z": 80.1875,
+ "stations": "Tani Base,Fourneyron Camp"
+ },
+ {
+ "name": "Bamini",
+ "pos_x": 98.5,
+ "pos_y": -50.0625,
+ "pos_z": 126.875,
+ "stations": "Frimout Reformatory,Rigaux Enterprise"
+ },
+ {
+ "name": "Baminyi",
+ "pos_x": 91.90625,
+ "pos_y": -110.34375,
+ "pos_z": -75.125,
+ "stations": "Glass Survey,Medupe Prospect,Flaugergues Penal colony"
+ },
+ {
+ "name": "Bamu",
+ "pos_x": -63.53125,
+ "pos_y": 20.46875,
+ "pos_z": 100.5,
+ "stations": "Tiedemann Dock,Spinrad Terminal,Fawcett Prospect,Atwood Point,Malchiodi Dock"
+ },
+ {
+ "name": "Bamum",
+ "pos_x": 115.34375,
+ "pos_y": 100.21875,
+ "pos_z": 67.4375,
+ "stations": "Bose Survey"
+ },
+ {
+ "name": "Bamuti",
+ "pos_x": -42.9375,
+ "pos_y": -99.125,
+ "pos_z": 60.75,
+ "stations": "Culpeper Terminal,Meikle Gateway,Feoktistov Orbital,Simpson Market,Bloomfield Ring,Agnesi Enterprise,Hart Port,Binnie Prospect,Blenkinsop Palace"
+ },
+ {
+ "name": "Bana",
+ "pos_x": -8.125,
+ "pos_y": -198.6875,
+ "pos_z": 46.21875,
+ "stations": "Esposito Enterprise,Hildebrandt Camp,Amundsen Silo"
+ },
+ {
+ "name": "Banacate",
+ "pos_x": -30.375,
+ "pos_y": -88.5,
+ "pos_z": 105.5,
+ "stations": "Al-Battani Settlement,Matteucci Port"
+ },
+ {
+ "name": "Banapityas",
+ "pos_x": 98.84375,
+ "pos_y": -2.84375,
+ "pos_z": 24.28125,
+ "stations": "Marshburn Dock,Treshchov Ring,Potter Hub,Yegorov Dock,Franklin Dock,Zahn Horizons,Tuan Port,Mitchell Gateway,Borlaug Station"
+ },
+ {
+ "name": "Banbara",
+ "pos_x": -38.3125,
+ "pos_y": -35.78125,
+ "pos_z": 105.03125,
+ "stations": "Mohmand Platform,Sinclair Platform"
+ },
+ {
+ "name": "Banbarulini",
+ "pos_x": 10.4375,
+ "pos_y": 91.5,
+ "pos_z": 114.75,
+ "stations": "Gubarev Prospect,Acton Prospect"
+ },
+ {
+ "name": "Banbudo",
+ "pos_x": 111.375,
+ "pos_y": -84.25,
+ "pos_z": -90.46875,
+ "stations": "Friend Orbital,Cousteau Port"
+ },
+ {
+ "name": "Banda",
+ "pos_x": -10.5,
+ "pos_y": -75.625,
+ "pos_z": 69.5,
+ "stations": "Poleshchuk Port,Lonchakov Station,Libby Orbital"
+ },
+ {
+ "name": "Bandizel",
+ "pos_x": 8,
+ "pos_y": -76.8125,
+ "pos_z": -35.09375,
+ "stations": "Hahn Orbital,Ryazanski City,Nikitin Hub,Hubble Hub,Great Hub"
+ },
+ {
+ "name": "Bandjigali",
+ "pos_x": -8.71875,
+ "pos_y": 7.0625,
+ "pos_z": 79.71875,
+ "stations": "Tayler Installation,Tull Orbital,Edison Station,Morin Terminal,Tombaugh Market,Russell Beacon,Teng-hui Station,Steiner Gateway,Manarov Settlement,Pavlov City"
+ },
+ {
+ "name": "Bandoque",
+ "pos_x": 120.6875,
+ "pos_y": -96.5,
+ "pos_z": -57.71875,
+ "stations": "Arfstrom Terminal"
+ },
+ {
+ "name": "Bandua",
+ "pos_x": 88.46875,
+ "pos_y": 9.78125,
+ "pos_z": 42.15625,
+ "stations": "Bosch Orbital,Gagnan Market"
+ },
+ {
+ "name": "Bandumar",
+ "pos_x": 85.5625,
+ "pos_y": -146.0625,
+ "pos_z": 119.59375,
+ "stations": "Kibalchich Platform,Adragna Survey,Nourse Relay"
+ },
+ {
+ "name": "Baneb Xoc",
+ "pos_x": -27.875,
+ "pos_y": 127.625,
+ "pos_z": 83.5625,
+ "stations": "Bramah Depot,Bennett Dock"
+ },
+ {
+ "name": "Banebdjetet",
+ "pos_x": 61.53125,
+ "pos_y": -25.625,
+ "pos_z": 80.9375,
+ "stations": "Mackenzie Dock,Maclaurin Installation"
+ },
+ {
+ "name": "Banei",
+ "pos_x": -62.3125,
+ "pos_y": 69.40625,
+ "pos_z": 108.1875,
+ "stations": "Tryggvason Stop"
+ },
+ {
+ "name": "Banek",
+ "pos_x": -75.75,
+ "pos_y": -143.4375,
+ "pos_z": -0.65625,
+ "stations": "Springer Orbital,Asire Observatory"
+ },
+ {
+ "name": "Banekalanec",
+ "pos_x": -19.40625,
+ "pos_y": 79.03125,
+ "pos_z": -59.0625,
+ "stations": "Resnick Platform,Coppel Prospect,Littlewood Platform"
+ },
+ {
+ "name": "Banemu",
+ "pos_x": -114.25,
+ "pos_y": 5.21875,
+ "pos_z": -0.5625,
+ "stations": "Ostwald Colony,Henize Station,Bamford Beacon"
+ },
+ {
+ "name": "Banephtha",
+ "pos_x": -82.8125,
+ "pos_y": -23.96875,
+ "pos_z": 140.8125,
+ "stations": "Lorrah Colony,Hoyle Survey,Butcher Settlement,Harding Reach,Chalker Point"
+ },
+ {
+ "name": "Banese",
+ "pos_x": -100.5,
+ "pos_y": -61.53125,
+ "pos_z": 98.84375,
+ "stations": "la Cosa Horizons,Tem Park,Sakers Hub"
+ },
+ {
+ "name": "Bang",
+ "pos_x": 34.34375,
+ "pos_y": -116.9375,
+ "pos_z": -49.21875,
+ "stations": "Piano Ring"
+ },
+ {
+ "name": "Bang Mu",
+ "pos_x": -77.71875,
+ "pos_y": -42.5,
+ "pos_z": 15.5,
+ "stations": "Salgari Dock"
+ },
+ {
+ "name": "Bangitani",
+ "pos_x": -13.375,
+ "pos_y": -12.5,
+ "pos_z": 149.96875,
+ "stations": "Wescott Dock,Ellison Settlement,Rozhdestvensky Platform"
+ },
+ {
+ "name": "Bango",
+ "pos_x": -56.90625,
+ "pos_y": 44.9375,
+ "pos_z": -81.125,
+ "stations": "Leiber Orbital,Hill Enterprise,Henry Point,Hausdorff Landing"
+ },
+ {
+ "name": "Bangputys",
+ "pos_x": -103.59375,
+ "pos_y": 126.59375,
+ "pos_z": -39.28125,
+ "stations": "Delany Legacy"
+ },
+ {
+ "name": "Bangwa",
+ "pos_x": 41.21875,
+ "pos_y": -3.46875,
+ "pos_z": 46.84375,
+ "stations": "Eanes Port,Rotsler Hub,Burnell Vista,McMullen Enterprise,Lefschetz Holdings"
+ },
+ {
+ "name": "Bangyani",
+ "pos_x": 37.78125,
+ "pos_y": -159.4375,
+ "pos_z": 101.34375,
+ "stations": "Staus Station,Kushida Enterprise,Dozois Relay,al-Khayyam Relay,Langford's Inheritance"
+ },
+ {
+ "name": "Banisas",
+ "pos_x": -97.34375,
+ "pos_y": 42.625,
+ "pos_z": -6.9375,
+ "stations": "Weyn Ring,Blaylock Port,McAllaster Hub"
+ },
+ {
+ "name": "Banji",
+ "pos_x": 134.21875,
+ "pos_y": -240.34375,
+ "pos_z": 85.125,
+ "stations": "Zwicky Beacon,Hipparchus Port,Crown Landing"
+ },
+ {
+ "name": "Banka",
+ "pos_x": -104.21875,
+ "pos_y": 122,
+ "pos_z": 40.59375,
+ "stations": "Alexander Escape,Hunt Colony"
+ },
+ {
+ "name": "Banki",
+ "pos_x": 49.53125,
+ "pos_y": 15.75,
+ "pos_z": -91.71875,
+ "stations": "Antonio de Andrade Vista,Diamond Port,Willis Laboratory,Escobar Holdings"
+ },
+ {
+ "name": "Bankoni",
+ "pos_x": 50.3125,
+ "pos_y": 13.90625,
+ "pos_z": 156.625,
+ "stations": "Oersted Beacon,Moskowitz Base"
+ },
+ {
+ "name": "Bankoponno",
+ "pos_x": -109.90625,
+ "pos_y": -167.5625,
+ "pos_z": 57.625,
+ "stations": "Noli's Exile"
+ },
+ {
+ "name": "Bany",
+ "pos_x": -60.125,
+ "pos_y": 68.71875,
+ "pos_z": 101.28125,
+ "stations": "Whymper Prospect,Weyl Depot"
+ },
+ {
+ "name": "Banya",
+ "pos_x": 40.84375,
+ "pos_y": 30.03125,
+ "pos_z": -99.0625,
+ "stations": "Peterson Platform"
+ },
+ {
+ "name": "Bao Yan Luo",
+ "pos_x": -31.40625,
+ "pos_y": -19.1875,
+ "pos_z": -42.53125,
+ "stations": "Behnken Terminal,Thuot Works"
+ },
+ {
+ "name": "Baracians",
+ "pos_x": 85.1875,
+ "pos_y": -196.84375,
+ "pos_z": -48.3125,
+ "stations": "Maury Terminal,Backlund Orbital,Korolev's Progress,Newcomb Station"
+ },
+ {
+ "name": "Barai",
+ "pos_x": -19.34375,
+ "pos_y": 163.1875,
+ "pos_z": 51.0625,
+ "stations": "Drzewiecki Dock,Janszoon Survey"
+ },
+ {
+ "name": "Baraluhet",
+ "pos_x": -29.40625,
+ "pos_y": -19.71875,
+ "pos_z": -119.125,
+ "stations": "Ziljak Outpost"
+ },
+ {
+ "name": "Baramanago",
+ "pos_x": 3.53125,
+ "pos_y": 126.53125,
+ "pos_z": -60.09375,
+ "stations": "Fremion Dock,Santos Dock,Reamy Survey,Jenkinson Point,Walker's Inheritance"
+ },
+ {
+ "name": "Baramat",
+ "pos_x": -24.875,
+ "pos_y": -122.625,
+ "pos_z": 35.09375,
+ "stations": "Burnham Orbital,Kludze Hub,Merritt Platform,Andoyer Vista"
+ },
+ {
+ "name": "Barangali",
+ "pos_x": 134.71875,
+ "pos_y": -230.40625,
+ "pos_z": 76.75,
+ "stations": "Nahavandi Port"
+ },
+ {
+ "name": "Barango",
+ "pos_x": -61.40625,
+ "pos_y": 36.15625,
+ "pos_z": 135.5,
+ "stations": "Gentle Vision,Roggeveen Horizons,Leavitt's Progress,Cartan Station,Plante Dock"
+ },
+ {
+ "name": "Barann",
+ "pos_x": 56.0625,
+ "pos_y": 60.84375,
+ "pos_z": 49.09375,
+ "stations": "Klimuk Refinery,Leibniz Hub,Crouch Silo"
+ },
+ {
+ "name": "Barasi",
+ "pos_x": 121.9375,
+ "pos_y": -77.96875,
+ "pos_z": -51.125,
+ "stations": "al-Kashi Orbital,Lyot Prospect,Ross' Progress"
+ },
+ {
+ "name": "Baraswar",
+ "pos_x": -11.375,
+ "pos_y": -36.59375,
+ "pos_z": 60.65625,
+ "stations": "Hoyle Lab,Walker Terminal"
+ },
+ {
+ "name": "Barathaona",
+ "pos_x": 84.15625,
+ "pos_y": 12.78125,
+ "pos_z": -1.875,
+ "stations": "Hermaszewski Gateway,Kregel Hub,Magnus Beacon,Sheckley Town,Thompson Orbital,Newton Enterprise,Beaufoy Barracks,Zudov Port,Taylor Orbital,Ejeta Dock,Dunyach Enterprise,Smith Port"
+ },
+ {
+ "name": "Barati",
+ "pos_x": 42.25,
+ "pos_y": 7.53125,
+ "pos_z": 71.5,
+ "stations": "Ryazanski Hub,Mastracchio Mines"
+ },
+ {
+ "name": "Barayai",
+ "pos_x": 178.8125,
+ "pos_y": -119.15625,
+ "pos_z": 33.375,
+ "stations": "Littrow Terminal,Bailly Port,Kurtz Colony,Stasheff's Folly"
+ },
+ {
+ "name": "Barbalalane",
+ "pos_x": -114.75,
+ "pos_y": -53,
+ "pos_z": -74.71875,
+ "stations": "Good Mines"
+ },
+ {
+ "name": "Barbaram",
+ "pos_x": 138.5,
+ "pos_y": -99.78125,
+ "pos_z": -45.78125,
+ "stations": "Simmons Settlement,Citi Orbital,Friesner Beacon,Vinge Settlement"
+ },
+ {
+ "name": "Bard",
+ "pos_x": -32.40625,
+ "pos_y": -136.15625,
+ "pos_z": 55.6875,
+ "stations": "Keeler Port,Fibonacci Vision,Arrhenius Terminal,Romer Orbital,Teng Refinery"
+ },
+ {
+ "name": "Bardgri",
+ "pos_x": 68.15625,
+ "pos_y": 110.46875,
+ "pos_z": 4.5625,
+ "stations": "Bohr Landing,Maxwell Hangar,Norgay Refinery"
+ },
+ {
+ "name": "Bare",
+ "pos_x": -104.40625,
+ "pos_y": 61.875,
+ "pos_z": 36.25,
+ "stations": "Glenn Relay,Mawson Horizons"
+ },
+ {
+ "name": "Barillian",
+ "pos_x": 121.3125,
+ "pos_y": 42.34375,
+ "pos_z": 12.625,
+ "stations": "Clayton Gateway,Rocklynne Observatory,Hardy Lab,Lie Barracks"
+ },
+ {
+ "name": "Baris",
+ "pos_x": 39.90625,
+ "pos_y": 35.8125,
+ "pos_z": 59.40625,
+ "stations": "Viktorenko Enterprise,Hartsfield Hub,Makarov Ring"
+ },
+ {
+ "name": "Bark Pular",
+ "pos_x": -43.375,
+ "pos_y": -54.125,
+ "pos_z": 79.84375,
+ "stations": "Anderson Terminal,Hardwick Colony,Gorbatko Settlement"
+ },
+ {
+ "name": "Barka",
+ "pos_x": 118.25,
+ "pos_y": -195.40625,
+ "pos_z": -19.59375,
+ "stations": "Tully Settlement,Froud Settlement,Tenn Beacon"
+ },
+ {
+ "name": "Barkan",
+ "pos_x": -58.53125,
+ "pos_y": 65.53125,
+ "pos_z": 44.1875,
+ "stations": "Harrison Port,Peano Hub,Collins Enterprise,Laplace Mines,Matthews Relay"
+ },
+ {
+ "name": "Barkindji",
+ "pos_x": -50.1875,
+ "pos_y": -87.5,
+ "pos_z": 34.84375,
+ "stations": "Fadlan Landing,Heaviside Refinery,Tryggvason Barracks,Henderson Holdings"
+ },
+ {
+ "name": "Barku",
+ "pos_x": 88.28125,
+ "pos_y": 68.875,
+ "pos_z": 17.28125,
+ "stations": "Avdeyev Station,Pudwill Gorie Port,Ocampo Survey,Kress Observatory"
+ },
+ {
+ "name": "Barnard's Star",
+ "pos_x": -3.03125,
+ "pos_y": 1.375,
+ "pos_z": 4.9375,
+ "stations": "Boston Base,Miller Depot,Kuttner's Pride,Levi-Strauss Installation,Haller City,Silves' Claim"
+ },
+ {
+ "name": "Baro",
+ "pos_x": -98.53125,
+ "pos_y": -140.9375,
+ "pos_z": 41.53125,
+ "stations": "Creamer Port,Berliner Gateway,Khrunov Colony,Dias Holdings"
+ },
+ {
+ "name": "Baroahy",
+ "pos_x": 96.46875,
+ "pos_y": 19.46875,
+ "pos_z": 106.46875,
+ "stations": "Treshchov Gateway,Parmitano Port,Piercy Vista,Popov Orbital"
+ },
+ {
+ "name": "Baroi",
+ "pos_x": 78.1875,
+ "pos_y": 40.625,
+ "pos_z": -83.53125,
+ "stations": "Dezhurov Landing"
+ },
+ {
+ "name": "Baroju",
+ "pos_x": -18.8125,
+ "pos_y": 46.4375,
+ "pos_z": -137.75,
+ "stations": "Soto Dock,Abasheli Dock,Lenthall Dock,Nansen Palace"
+ },
+ {
+ "name": "Baroo",
+ "pos_x": 164.1875,
+ "pos_y": -172.84375,
+ "pos_z": -24.53125,
+ "stations": "Hampson Hub,Heinemann Station,Sheckley Town,Stefansson Port"
+ },
+ {
+ "name": "Barotrites",
+ "pos_x": 137.28125,
+ "pos_y": -71.1875,
+ "pos_z": 86.28125,
+ "stations": "Horrocks Vision,Schachner Arsenal"
+ },
+ {
+ "name": "Baruai Shi",
+ "pos_x": -54.78125,
+ "pos_y": -64.0625,
+ "pos_z": 60,
+ "stations": "Lazutkin Port"
+ },
+ {
+ "name": "Barueker",
+ "pos_x": -116.78125,
+ "pos_y": 56.84375,
+ "pos_z": -119.5625,
+ "stations": "Reynolds Depot"
+ },
+ {
+ "name": "Baruga",
+ "pos_x": -17.84375,
+ "pos_y": -15.5625,
+ "pos_z": -176.34375,
+ "stations": "Shunn Port,Tanner Mine,Lee Enterprise"
+ },
+ {
+ "name": "Barumbo",
+ "pos_x": 76.40625,
+ "pos_y": 79.90625,
+ "pos_z": 33.875,
+ "stations": "Brash Refinery"
+ },
+ {
+ "name": "Barundjal",
+ "pos_x": 129.1875,
+ "pos_y": -149.59375,
+ "pos_z": 31.96875,
+ "stations": "Zacuto Port,Mayer Port,Gold Terminal,Gentil Vision"
+ },
+ {
+ "name": "Basi",
+ "pos_x": 99.25,
+ "pos_y": 18.03125,
+ "pos_z": -44.3125,
+ "stations": "Tanner Base,Ryman Vision"
+ },
+ {
+ "name": "Basigi",
+ "pos_x": -78.21875,
+ "pos_y": -53.59375,
+ "pos_z": -89.875,
+ "stations": "Pausch Horizons,Pauli Horizons,Feynman Holdings,Janifer Bastion,Searfoss Installation"
+ },
+ {
+ "name": "Basika",
+ "pos_x": -32.875,
+ "pos_y": 20,
+ "pos_z": 83.71875,
+ "stations": "Howard City,Bohm Hangar"
+ },
+ {
+ "name": "Basikasingo",
+ "pos_x": 122.90625,
+ "pos_y": -112.1875,
+ "pos_z": 117.8125,
+ "stations": "Alphonsi Depot"
+ },
+ {
+ "name": "Basile",
+ "pos_x": -111.59375,
+ "pos_y": 11.25,
+ "pos_z": 51.21875,
+ "stations": "Verrazzano Platform,Grimwood Hub,Surayev's Claim"
+ },
+ {
+ "name": "Basing",
+ "pos_x": -95.9375,
+ "pos_y": -72.125,
+ "pos_z": -111.84375,
+ "stations": "Dzhanibekov Landing,Fisher Works,Cartier Refinery"
+ },
+ {
+ "name": "Bassa",
+ "pos_x": -84.75,
+ "pos_y": -44.8125,
+ "pos_z": -132.3125,
+ "stations": "Dayuan City"
+ },
+ {
+ "name": "Basses",
+ "pos_x": -77.34375,
+ "pos_y": 11.5,
+ "pos_z": 123.53125,
+ "stations": "Robinson Settlement"
+ },
+ {
+ "name": "Bast",
+ "pos_x": -36.46875,
+ "pos_y": 16.15625,
+ "pos_z": -34.9375,
+ "stations": "Hart Station"
+ },
+ {
+ "name": "Bastae",
+ "pos_x": 121.375,
+ "pos_y": 97.21875,
+ "pos_z": 19,
+ "stations": "Lynn Point"
+ },
+ {
+ "name": "Bastan",
+ "pos_x": 6.4375,
+ "pos_y": 33.5625,
+ "pos_z": 94.75,
+ "stations": "Tesla Refinery"
+ },
+ {
+ "name": "Bastana",
+ "pos_x": 68.0625,
+ "pos_y": 6.75,
+ "pos_z": -159.65625,
+ "stations": "Lopez de Legazpi Enterprise,Menezes Orbital,Wilson Port,Wilson Base"
+ },
+ {
+ "name": "Bastanien",
+ "pos_x": 161.25,
+ "pos_y": -100.1875,
+ "pos_z": -17.4375,
+ "stations": "Sweet Port,Shaw Colony"
+ },
+ {
+ "name": "Bastas",
+ "pos_x": -99.53125,
+ "pos_y": -121.28125,
+ "pos_z": -21.28125,
+ "stations": "Steiner Terminal,Anthony Dock"
+ },
+ {
+ "name": "Basterni",
+ "pos_x": 111.25,
+ "pos_y": -257.84375,
+ "pos_z": 52.75,
+ "stations": "Mikulin Hub,Kuchemann Terminal,Perlmutter Terminal,Goodman Beacon,Blish Depot"
+ },
+ {
+ "name": "Bastes",
+ "pos_x": -44.90625,
+ "pos_y": 46.1875,
+ "pos_z": 96.5625,
+ "stations": "Shepherd Hub,Tyurin Settlement,Kelly Settlement"
+ },
+ {
+ "name": "Bastsegezi",
+ "pos_x": 86.34375,
+ "pos_y": 12.75,
+ "pos_z": -113.5,
+ "stations": "Phillpotts Enterprise,Poncelet Enterprise,Larson Terminal,Sterling Base,Abbott Laboratory"
+ },
+ {
+ "name": "Basty",
+ "pos_x": -38.4375,
+ "pos_y": 79.0625,
+ "pos_z": 109.875,
+ "stations": "Carver Relay,Russell Sanctuary"
+ },
+ {
+ "name": "Basuk",
+ "pos_x": 34.375,
+ "pos_y": -135.03125,
+ "pos_z": 111.78125,
+ "stations": "Urata Colony,de Seversky Dock,Kettle Depot,Tomita Plant"
+ },
+ {
+ "name": "Basuki An",
+ "pos_x": 20,
+ "pos_y": -103.4375,
+ "pos_z": -89.40625,
+ "stations": "Jacobi Hub,Shinn Bastion,Cabral Lab"
+ },
+ {
+ "name": "Basus",
+ "pos_x": -95.65625,
+ "pos_y": 38.0625,
+ "pos_z": -13.46875,
+ "stations": "Carpenter Landing,Ramanujan Orbital,Wallace Port,Dall's Progress,Vasyutin's Progress"
+ },
+ {
+ "name": "Basuseku",
+ "pos_x": 158.34375,
+ "pos_y": -201.96875,
+ "pos_z": 49.125,
+ "stations": "Apianus Dock,Sei Landing,Kopal Survey,Blair's Inheritance,Lindbohm Market"
+ },
+ {
+ "name": "Bataenich",
+ "pos_x": 97.0625,
+ "pos_y": -141.125,
+ "pos_z": -10.8125,
+ "stations": "de Seversky Port,Tuttle Terminal,Roskam Mines,Desargues' Claim"
+ },
+ {
+ "name": "Batani",
+ "pos_x": -134.03125,
+ "pos_y": 57.34375,
+ "pos_z": -26.53125,
+ "stations": "Linnehan Base,Curbeam's Claim"
+ },
+ {
+ "name": "Batar",
+ "pos_x": 97.78125,
+ "pos_y": 99.34375,
+ "pos_z": -81.65625,
+ "stations": "Budarin Camp"
+ },
+ {
+ "name": "Batara Gura",
+ "pos_x": -91.8125,
+ "pos_y": 25.40625,
+ "pos_z": -129.15625,
+ "stations": "Libby Horizons,Gelfand Horizons,Mastracchio Settlement"
+ },
+ {
+ "name": "Batari",
+ "pos_x": -139.71875,
+ "pos_y": 109.28125,
+ "pos_z": 15.3125,
+ "stations": "Niven Ring,Chandler Hub,Alpers Vista,Popov Terminal,Lopez de Legazpi Settlement"
+ },
+ {
+ "name": "Batho",
+ "pos_x": -55.9375,
+ "pos_y": -103,
+ "pos_z": -57.09375,
+ "stations": "Doyle Dock,Riemann Plant,Gibson Freeport"
+ },
+ {
+ "name": "Batjan",
+ "pos_x": -123.25,
+ "pos_y": -19.1875,
+ "pos_z": 52.46875,
+ "stations": "Smith Landing,Wrangel Relay,Sinisalo Penal colony"
+ },
+ {
+ "name": "Batz",
+ "pos_x": -23.1875,
+ "pos_y": -59.53125,
+ "pos_z": 40.625,
+ "stations": "Krylov's Inheritance,Bose Settlement,Lamarr Holdings"
+ },
+ {
+ "name": "Batzin",
+ "pos_x": 190.0625,
+ "pos_y": -141.84375,
+ "pos_z": 70.5625,
+ "stations": "von Zach Ring,Leonard City,Penzias Prospect,Jenkinson Works,Wells Beacon"
+ },
+ {
+ "name": "Baudani",
+ "pos_x": -80.71875,
+ "pos_y": -125.1875,
+ "pos_z": -99.21875,
+ "stations": "Simonyi Orbital,Cassidy Beacon,Swanwick Landing"
+ },
+ {
+ "name": "Baudhea",
+ "pos_x": 95.125,
+ "pos_y": -156.65625,
+ "pos_z": -13.21875,
+ "stations": "Fieseler Base,Maury Colony,Grigorovich Vision"
+ },
+ {
+ "name": "Baudihe",
+ "pos_x": 163.84375,
+ "pos_y": -111.96875,
+ "pos_z": -33.03125,
+ "stations": "Ghez Mines,Mason Dock,Wallerstein Horizons"
+ },
+ {
+ "name": "Baudiko O",
+ "pos_x": 82.8125,
+ "pos_y": -99.65625,
+ "pos_z": 136.40625,
+ "stations": "Melia Beacon"
+ },
+ {
+ "name": "Baudu",
+ "pos_x": 39.46875,
+ "pos_y": -23.9375,
+ "pos_z": -19.09375,
+ "stations": "Wilhelm Refinery,Moore Installation,Noli Settlement,Jenkinson Vision"
+ },
+ {
+ "name": "Baudugari",
+ "pos_x": 163.90625,
+ "pos_y": -122.21875,
+ "pos_z": 111.4375,
+ "stations": "Baily Vision"
+ },
+ {
+ "name": "Baudurotri",
+ "pos_x": 60.53125,
+ "pos_y": -166.25,
+ "pos_z": 172.78125,
+ "stations": "Wilhelm von Struve Orbital,Key Silo,Hall Holdings,Lockwood Station,al-Khayyam Vision"
+ },
+ {
+ "name": "Baudus",
+ "pos_x": -68.09375,
+ "pos_y": 41.0625,
+ "pos_z": -30.96875,
+ "stations": "Rice Dock,Effinger Point,Phillpotts Terminal"
+ },
+ {
+ "name": "Bava",
+ "pos_x": 83.40625,
+ "pos_y": -134.3125,
+ "pos_z": -80.75,
+ "stations": "Bauschinger City,Wallis Holdings"
+ },
+ {
+ "name": "Bavapri",
+ "pos_x": 111.78125,
+ "pos_y": -240.125,
+ "pos_z": 19.53125,
+ "stations": "Walotsky Sanctuary"
+ },
+ {
+ "name": "Bavarigga",
+ "pos_x": -76.46875,
+ "pos_y": 113.4375,
+ "pos_z": -35.46875,
+ "stations": "Hippalus Prospect,Shaver Stop"
+ },
+ {
+ "name": "Bavaringoni",
+ "pos_x": -34.65625,
+ "pos_y": -26.65625,
+ "pos_z": 47.6875,
+ "stations": "Chandler Platform"
+ },
+ {
+ "name": "Baxbakaeris",
+ "pos_x": 18.625,
+ "pos_y": -12.375,
+ "pos_z": -16.46875,
+ "stations": "Beaumont Installation,Stillman Station,Burkin Hub,Yurchikhin Hub,Nansen Enterprise"
+ },
+ {
+ "name": "Baxbalabia",
+ "pos_x": -91.34375,
+ "pos_y": 72.25,
+ "pos_z": -68.15625,
+ "stations": "Bamford Holdings,Herreshoff Beacon,Edwards Holdings"
+ },
+ {
+ "name": "Baya",
+ "pos_x": 146,
+ "pos_y": -30.75,
+ "pos_z": 29.84375,
+ "stations": "McKean Orbital,MacLeod Port"
+ },
+ {
+ "name": "Bayah",
+ "pos_x": 25.75,
+ "pos_y": -189.15625,
+ "pos_z": -55.625,
+ "stations": "Mobius Enterprise,Wallis Vision,Riemann Hub"
+ },
+ {
+ "name": "Bayahutina",
+ "pos_x": -23.84375,
+ "pos_y": -146.625,
+ "pos_z": 111.90625,
+ "stations": "Swanwick Landing,Tudela Works,Dampier Hub"
+ },
+ {
+ "name": "Bayan",
+ "pos_x": -19.96875,
+ "pos_y": 117.625,
+ "pos_z": -90.46875,
+ "stations": "Bloch Platform,Lewis Dock,Kronecker Reach"
+ },
+ {
+ "name": "BD+00 197",
+ "pos_x": -36.4375,
+ "pos_y": -93.53125,
+ "pos_z": -36.71875,
+ "stations": "Nourse Horizons"
+ },
+ {
+ "name": "BD+01 299",
+ "pos_x": -31.78125,
+ "pos_y": -96.71875,
+ "pos_z": -50.75,
+ "stations": "Platt Plant"
+ },
+ {
+ "name": "BD+01 3574",
+ "pos_x": -54.25,
+ "pos_y": 21.09375,
+ "pos_z": 96.125,
+ "stations": "Savinykh Station,Bottego Arsenal"
+ },
+ {
+ "name": "BD+01 3657",
+ "pos_x": -45.34375,
+ "pos_y": 10.21875,
+ "pos_z": 74.125,
+ "stations": "Ham Settlement,Sacco Base"
+ },
+ {
+ "name": "BD+02 305",
+ "pos_x": -8.90625,
+ "pos_y": -28.84375,
+ "pos_z": -17.90625,
+ "stations": "Swainson Station"
+ },
+ {
+ "name": "BD+02 4718",
+ "pos_x": -46.4375,
+ "pos_y": -69.59375,
+ "pos_z": -4.375,
+ "stations": "Cummings Terminal,Abernathy Enterprise,Celebi Horizons"
+ },
+ {
+ "name": "BD+03 1552",
+ "pos_x": 45.15625,
+ "pos_y": 8.34375,
+ "pos_z": -72.25,
+ "stations": "Narita Ring,Bailey Station"
+ },
+ {
+ "name": "BD+03 2279",
+ "pos_x": 40.9375,
+ "pos_y": 44.125,
+ "pos_z": -28.84375,
+ "stations": "Vespucci Penal colony,Smith Hub,Ballard Vision"
+ },
+ {
+ "name": "BD+03 2316",
+ "pos_x": 44.71875,
+ "pos_y": 51.34375,
+ "pos_z": -27.875,
+ "stations": "den Berg Ring,Gooch Terminal,Bloomfield Hub,Baudry Enterprise,Usachov Terminal"
+ },
+ {
+ "name": "BD+03 2338",
+ "pos_x": 69.28125,
+ "pos_y": 82.78125,
+ "pos_z": -41.03125,
+ "stations": "Hudson Dock,Dayuan Vision"
+ },
+ {
+ "name": "BD+03 3531a",
+ "pos_x": -41.78125,
+ "pos_y": 20.125,
+ "pos_z": 71.875,
+ "stations": "Zhen City,Morgan Dock,Phillifent Enterprise,Horowitz Hub,Ponce de Leon Depot"
+ },
+ {
+ "name": "BD+03 515",
+ "pos_x": 3.3125,
+ "pos_y": -48.53125,
+ "pos_z": -61.53125,
+ "stations": "Walz Survey,Priestley's Claim"
+ },
+ {
+ "name": "BD+04 4344",
+ "pos_x": -80.625,
+ "pos_y": -28.46875,
+ "pos_z": 75.40625,
+ "stations": "Sacco Hub,Reilly City,Monge Survey,Vela Hub"
+ },
+ {
+ "name": "BD+04 4988",
+ "pos_x": -52.6875,
+ "pos_y": -63.21875,
+ "pos_z": 4.03125,
+ "stations": "Sterling Point"
+ },
+ {
+ "name": "BD+05 1146",
+ "pos_x": 41.03125,
+ "pos_y": -9.15625,
+ "pos_z": -90.53125,
+ "stations": "Boyle Orbital,Ellis Gateway,Kingsbury Settlement,Melvin Dock,Ron Hubbard Holdings,Meade Hub,Musabayev City,Grunsfeld Ring,Salak Point,Hilbert Hub"
+ },
+ {
+ "name": "BD+05 1295",
+ "pos_x": 50.34375,
+ "pos_y": -1.9375,
+ "pos_z": -101.78125,
+ "stations": "Scott Orbital,Levi-Montalcini Port,Lambert Holdings"
+ },
+ {
+ "name": "BD+05 2131",
+ "pos_x": 44.03125,
+ "pos_y": 40.375,
+ "pos_z": -43.875,
+ "stations": "Stevens Enterprise"
+ },
+ {
+ "name": "BD+05 2950",
+ "pos_x": -2.875,
+ "pos_y": 82.96875,
+ "pos_z": 65.21875,
+ "stations": "Malerba Plant"
+ },
+ {
+ "name": "BD+05 5244",
+ "pos_x": -47.6875,
+ "pos_y": -66.875,
+ "pos_z": -10.09375,
+ "stations": "Hoshi Hangar,Fidalgo Landing,Babakin Installation"
+ },
+ {
+ "name": "BD+06 5034",
+ "pos_x": -78.5625,
+ "pos_y": -73.46875,
+ "pos_z": 21.71875,
+ "stations": "Descartes Hub,Davis Landing"
+ },
+ {
+ "name": "BD+07 1919",
+ "pos_x": 61.90625,
+ "pos_y": 40.4375,
+ "pos_z": -87.25,
+ "stations": "Silves Prospect,Cook Platform"
+ },
+ {
+ "name": "BD+07 2008",
+ "pos_x": 45.84375,
+ "pos_y": 37.46875,
+ "pos_z": -55.25,
+ "stations": "Sarrantonio Ring,Beatty Terminal,Houtman Orbital"
+ },
+ {
+ "name": "BD+07 2869",
+ "pos_x": -6.25,
+ "pos_y": 91.40625,
+ "pos_z": 68.1875,
+ "stations": "Deb Vision,Moorcock Reach"
+ },
+ {
+ "name": "BD+07 4267",
+ "pos_x": -82.5,
+ "pos_y": -20.59375,
+ "pos_z": 76.65625,
+ "stations": "Rorschach Survey"
+ },
+ {
+ "name": "BD+08 1303",
+ "pos_x": 25.53125,
+ "pos_y": -1.875,
+ "pos_z": -62.84375,
+ "stations": "Chomsky Ring,Wescott Terminal"
+ },
+ {
+ "name": "BD+08 3000",
+ "pos_x": -12.78125,
+ "pos_y": 85.625,
+ "pos_z": 70.59375,
+ "stations": "Bernoulli Installation,Tokubei Orbital,Langford Plant"
+ },
+ {
+ "name": "BD+08 642",
+ "pos_x": 4.90625,
+ "pos_y": -49.1875,
+ "pos_z": -88.1875,
+ "stations": "Schmitt Platform,Velazquez's Progress"
+ },
+ {
+ "name": "BD+09 1105",
+ "pos_x": 36.40625,
+ "pos_y": -8.3125,
+ "pos_z": -100.5625,
+ "stations": "Shargin Port,Citroen Horizons"
+ },
+ {
+ "name": "BD+09 3000",
+ "pos_x": -11.5,
+ "pos_y": 81.78125,
+ "pos_z": 61.375,
+ "stations": "Hire Mines,Hertz Colony,Larson Installation"
+ },
+ {
+ "name": "BD+10 3250",
+ "pos_x": -60.65625,
+ "pos_y": 40.71875,
+ "pos_z": 88.5625,
+ "stations": "Narita Depot,Vetulani Installation,Lichtenberg Station"
+ },
+ {
+ "name": "BD+10 4385",
+ "pos_x": -89.5,
+ "pos_y": -38.71875,
+ "pos_z": 55.15625,
+ "stations": "Maybury Beacon,Lopez de Haro Terminal,Baker Penal colony"
+ },
+ {
+ "name": "BD+10 5022",
+ "pos_x": -63.21875,
+ "pos_y": -77.90625,
+ "pos_z": -17,
+ "stations": "Malenchenko Hangar,Stanier Hub,Leinster Prospect"
+ },
+ {
+ "name": "BD+11 2673",
+ "pos_x": -1.65625,
+ "pos_y": 103.625,
+ "pos_z": 54.03125,
+ "stations": "Luiken Orbital,Nagata City,Paulo da Gama Hub,Wisniewski-Snerg City,Kronecker Port"
+ },
+ {
+ "name": "BD+11 2811",
+ "pos_x": -15.625,
+ "pos_y": 63.21875,
+ "pos_z": 52.40625,
+ "stations": "Nobel Vision"
+ },
+ {
+ "name": "BD+11 2853",
+ "pos_x": -26.09375,
+ "pos_y": 77,
+ "pos_z": 70.0625,
+ "stations": "Ostwald Base,Midgeley Settlement,Nicolet Enterprise,Rustah Works"
+ },
+ {
+ "name": "BD+11 2925",
+ "pos_x": -35.625,
+ "pos_y": 73.15625,
+ "pos_z": 77.75,
+ "stations": "Zajdel Terminal,Fuchs Ring,Taylor Hub"
+ },
+ {
+ "name": "BD+11 570",
+ "pos_x": 0.28125,
+ "pos_y": -55.0625,
+ "pos_z": -102.875,
+ "stations": "Forrester Dock,Landsteiner Outpost"
+ },
+ {
+ "name": "BD+12 1759",
+ "pos_x": 50.5625,
+ "pos_y": 40.9375,
+ "pos_z": -86.5625,
+ "stations": "Ivanov Beacon,Perrin Port"
+ },
+ {
+ "name": "BD+12 5016",
+ "pos_x": -74.625,
+ "pos_y": -78.9375,
+ "pos_z": -12.34375,
+ "stations": "Johnson Vision"
+ },
+ {
+ "name": "BD+12 810",
+ "pos_x": 24.9375,
+ "pos_y": -21.875,
+ "pos_z": -111.625,
+ "stations": "Dyson Horizons,Brandenstein Hub,Issigonis Vision"
+ },
+ {
+ "name": "BD+13 3769",
+ "pos_x": -83.875,
+ "pos_y": 12.28125,
+ "pos_z": 84.28125,
+ "stations": "Bixby Camp,Berners-Lee Settlement,Furrer Dock"
+ },
+ {
+ "name": "BD+13 693",
+ "pos_x": 4.96875,
+ "pos_y": -42.875,
+ "pos_z": -106.625,
+ "stations": "Drummond's Progress"
+ },
+ {
+ "name": "BD+14 1876",
+ "pos_x": 35.71875,
+ "pos_y": 35.4375,
+ "pos_z": -61.0625,
+ "stations": "Leestma Enterprise,Pirsan Terminal,Niven Prospect,Hawley Enterprise,Agassiz Vision"
+ },
+ {
+ "name": "BD+14 2955",
+ "pos_x": -34.1875,
+ "pos_y": 78.78125,
+ "pos_z": 70.84375,
+ "stations": "Wisoff Base"
+ },
+ {
+ "name": "BD+14 4559",
+ "pos_x": -131.625,
+ "pos_y": -61.5,
+ "pos_z": 61.40625,
+ "stations": "Meitner Vision,Fernandes de Queiros Point"
+ },
+ {
+ "name": "BD+14 611",
+ "pos_x": -9.03125,
+ "pos_y": -49.78125,
+ "pos_z": -88.96875,
+ "stations": "Celsius Point,Jahn Plant"
+ },
+ {
+ "name": "BD+14 831",
+ "pos_x": 9.90625,
+ "pos_y": -19.8125,
+ "pos_z": -74,
+ "stations": "Strekalov Prospect,Kondakova Orbital"
+ },
+ {
+ "name": "BD+15 176",
+ "pos_x": -49.90625,
+ "pos_y": -68.34375,
+ "pos_z": -44.28125,
+ "stations": "Grechko Hub,Mohmand Enterprise,Dunn Dock,Stott Ring,Walter Hub,Virchow Port,Chun Installation,Martinez Relay,Hope Enterprise,Gamow Gateway,Kavandi Hub,Hornby Relay"
+ },
+ {
+ "name": "BD+15 2847",
+ "pos_x": -22.6875,
+ "pos_y": 79.8125,
+ "pos_z": 55.96875,
+ "stations": "Reynolds Terminal,Kaleri Gateway,Halley Port,Melvill Landing"
+ },
+ {
+ "name": "BD+15 3090",
+ "pos_x": -58.1875,
+ "pos_y": 60.34375,
+ "pos_z": 83.15625,
+ "stations": "Kizim Terminal,Harvey Enterprise,Yang Enterprise"
+ },
+ {
+ "name": "BD+15 4416",
+ "pos_x": -92.125,
+ "pos_y": -45.1875,
+ "pos_z": 36.3125,
+ "stations": "Debye Hub,Williams Silo,Emshwiller Relay"
+ },
+ {
+ "name": "BD+15 4829",
+ "pos_x": -86.875,
+ "pos_y": -79.6875,
+ "pos_z": -9.75,
+ "stations": "Kimbrough Terminal,Martinez Landing"
+ },
+ {
+ "name": "BD+15 896",
+ "pos_x": 23.53125,
+ "pos_y": -15.09375,
+ "pos_z": -114.6875,
+ "stations": "Hawking Terminal,Hoard Hub"
+ },
+ {
+ "name": "BD+16 1001",
+ "pos_x": 26.9375,
+ "pos_y": -2.71875,
+ "pos_z": -112,
+ "stations": "Bessemer Terminal"
+ },
+ {
+ "name": "BD+16 2404",
+ "pos_x": 8.875,
+ "pos_y": 45.1875,
+ "pos_z": 3.9375,
+ "stations": "Barba Terminal"
+ },
+ {
+ "name": "BD+16 2722",
+ "pos_x": -17.71875,
+ "pos_y": 83.125,
+ "pos_z": 50.46875,
+ "stations": "Robinson Landing,Hooke Camp,Hottot Arsenal"
+ },
+ {
+ "name": "BD+17 4808",
+ "pos_x": -71.8125,
+ "pos_y": -51.375,
+ "pos_z": 4.03125,
+ "stations": "Doi Works,Lichtenberg Landing,Fowler Keep"
+ },
+ {
+ "name": "BD+18 1160",
+ "pos_x": 25.125,
+ "pos_y": 2.8125,
+ "pos_z": -105.09375,
+ "stations": "Voss Camp,Lorentz Survey,Alpers Camp"
+ },
+ {
+ "name": "BD+18 1461",
+ "pos_x": 33.34375,
+ "pos_y": 20.8125,
+ "pos_z": -99.25,
+ "stations": "Onizuka Colony,O'Leary Base"
+ },
+ {
+ "name": "BD+19 2443",
+ "pos_x": 30.375,
+ "pos_y": 93.84375,
+ "pos_z": -22.875,
+ "stations": "Plante Colony,Clifford Dock,Malcolm Mines,Nachtigal Enterprise"
+ },
+ {
+ "name": "BD+19 2511",
+ "pos_x": 20.75,
+ "pos_y": 82.75,
+ "pos_z": -10.375,
+ "stations": "Lie Terminal,Okorafor Orbital,Poncelet's Inheritance"
+ },
+ {
+ "name": "BD+19 2929",
+ "pos_x": -27.6875,
+ "pos_y": 96.96875,
+ "pos_z": 56.78125,
+ "stations": "Wilcutt Enterprise,Chun Point,Murphy Point"
+ },
+ {
+ "name": "BD+20 1018",
+ "pos_x": 12,
+ "pos_y": -10.28125,
+ "pos_z": -108.6875,
+ "stations": "Maury Vision,Popov Vision"
+ },
+ {
+ "name": "BD+20 518",
+ "pos_x": -46.28125,
+ "pos_y": -88.59375,
+ "pos_z": -142.84375,
+ "stations": "Christopher Dock"
+ },
+ {
+ "name": "BD+21 1952",
+ "pos_x": 41.03125,
+ "pos_y": 71.03125,
+ "pos_z": -82.46875,
+ "stations": "Offutt Base,Ramelli Port"
+ },
+ {
+ "name": "BD+21 55",
+ "pos_x": -75.53125,
+ "pos_y": -70.53125,
+ "pos_z": -39.28125,
+ "stations": "Pinzon Hub,Roberts Terminal,Fontenay Gateway,Watts City,Keyes Terminal,Bushkov Enterprise,Lagrange Hub"
+ },
+ {
+ "name": "BD+22 2271",
+ "pos_x": 31.4375,
+ "pos_y": 94.40625,
+ "pos_z": -39.8125,
+ "stations": "Armstrong Survey,Wankel Station,Newman Enterprise,Harness Terminal,Hire Barracks"
+ },
+ {
+ "name": "BD+22 2632",
+ "pos_x": -4.90625,
+ "pos_y": 88.4375,
+ "pos_z": 22.53125,
+ "stations": "Altshuller Relay,Ellern Ring,Moran Platform,Puleston Bastion,Scheerbart Colony"
+ },
+ {
+ "name": "BD+22 2742",
+ "pos_x": -17.875,
+ "pos_y": 75.65625,
+ "pos_z": 33.40625,
+ "stations": "Wellman Port,Levi-Montalcini Orbital,Cunningham Hub,Young Prospect,Ellis Dock,Rontgen Dock,Hope Terminal,Perrin Vision,Whitney Port,Garriott City,Garratt Orbital,Khayyam Installation"
+ },
+ {
+ "name": "BD+22 3579",
+ "pos_x": -84.6875,
+ "pos_y": 12.71875,
+ "pos_z": 58.4375,
+ "stations": "Gerst Ring,Alcala Dock,Morris Landing,Grandin Point"
+ },
+ {
+ "name": "BD+22 4939",
+ "pos_x": -235.90625,
+ "pos_y": -192.84375,
+ "pos_z": -80.46875,
+ "stations": "Hansford's Landing,Ingstad Base,Dann Landing"
+ },
+ {
+ "name": "BD+23 204",
+ "pos_x": -62.53125,
+ "pos_y": -68.875,
+ "pos_z": -64.28125,
+ "stations": "Potrykus Holdings,Geston Installation"
+ },
+ {
+ "name": "BD+23 2640",
+ "pos_x": -8.25,
+ "pos_y": 77.53125,
+ "pos_z": 21.46875,
+ "stations": "Humphreys Station,Wagner Dock,Vasquez de Coronado Horizons,Zebrowski Hub"
+ },
+ {
+ "name": "BD+23 296",
+ "pos_x": -54.40625,
+ "pos_y": -68.28125,
+ "pos_z": -81.59375,
+ "stations": "Debye Gateway"
+ },
+ {
+ "name": "BD+23 3035",
+ "pos_x": -55.125,
+ "pos_y": 52.15625,
+ "pos_z": 57.6875,
+ "stations": "Kerwin Platform"
+ },
+ {
+ "name": "BD+23 3151",
+ "pos_x": -47.65625,
+ "pos_y": 31,
+ "pos_z": 44.59375,
+ "stations": "Bamford Ring,Carr Base,de Sousa Penal colony,Houssay Port,McCoy Terminal,Kaleri Vision,Fu Laboratory,Otto Dock"
+ },
+ {
+ "name": "BD+23 4334",
+ "pos_x": -107.71875,
+ "pos_y": -40.625,
+ "pos_z": 27.1875,
+ "stations": "King Depot,Lerman Keep,Chiang Colony"
+ },
+ {
+ "name": "BD+24 1451",
+ "pos_x": 19.4375,
+ "pos_y": 20.40625,
+ "pos_z": -93.40625,
+ "stations": "Marshburn Terminal,Mayr Ring,Sabine Point,Meade Dock,Bouch Base"
+ },
+ {
+ "name": "BD+24 2539",
+ "pos_x": 0.90625,
+ "pos_y": 114.9375,
+ "pos_z": 10.8125,
+ "stations": "Nolan Estate,Kummer Port,Tshang Installation"
+ },
+ {
+ "name": "BD+24 543",
+ "pos_x": -14.53125,
+ "pos_y": -25.125,
+ "pos_z": -56.3125,
+ "stations": "Katzenstein Settlement,Proteus Orbital"
+ },
+ {
+ "name": "BD+26 2184",
+ "pos_x": 13.21875,
+ "pos_y": 64.21875,
+ "pos_z": -21.0625,
+ "stations": "Grover Hub,Pailes Depot,Wiberg Hub,Garn Hub,Torricelli Hub"
+ },
+ {
+ "name": "BD+26 2723",
+ "pos_x": -44.71875,
+ "pos_y": 85.5,
+ "pos_z": 50.09375,
+ "stations": "Vinogradov Colony,Cameron Horizons,Alexandria Relay"
+ },
+ {
+ "name": "BD+26 8",
+ "pos_x": -65.03125,
+ "pos_y": -49.0625,
+ "pos_z": -27.59375,
+ "stations": "Merbold Hub,Margulis Base"
+ },
+ {
+ "name": "BD+27 1356",
+ "pos_x": 20.03125,
+ "pos_y": 34.09375,
+ "pos_z": -101.9375,
+ "stations": "Bester Holdings,Whitson Platform,Birkeland Dock"
+ },
+ {
+ "name": "BD+27 1739",
+ "pos_x": 15.53125,
+ "pos_y": 42.78125,
+ "pos_z": -43.15625,
+ "stations": "Buffett Ring,Dyomin Terminal,Hoshide Survey,Jolliet Point"
+ },
+ {
+ "name": "BD+27 881",
+ "pos_x": 4.875,
+ "pos_y": 0.90625,
+ "pos_z": -118.25,
+ "stations": "Sherrington Outpost,Redi Settlement,Cartmill Hub"
+ },
+ {
+ "name": "BD+28 2272",
+ "pos_x": -14.4375,
+ "pos_y": 91.84375,
+ "pos_z": 18.1875,
+ "stations": "Ellison Enterprise"
+ },
+ {
+ "name": "BD+28 413",
+ "pos_x": -54.125,
+ "pos_y": -56.09375,
+ "pos_z": -84.28125,
+ "stations": "Gunn Station,Diophantus Enterprise,Holub Survey,Hiroyuki Prospect"
+ },
+ {
+ "name": "BD+28 4660",
+ "pos_x": -59.03125,
+ "pos_y": -38.96875,
+ "pos_z": -19.875,
+ "stations": "Thurston Relay,Somerset Enterprise,Walker Orbital,Szameit Observatory,Taylor Hub"
+ },
+ {
+ "name": "BD+29 1754",
+ "pos_x": 22.65625,
+ "pos_y": 57.84375,
+ "pos_z": -85.96875,
+ "stations": "Carlisle Hub,Sakers Legacy"
+ },
+ {
+ "name": "BD+29 2405",
+ "pos_x": -6.28125,
+ "pos_y": 60.8125,
+ "pos_z": 5.5,
+ "stations": "Hasse Prospect,Benyovszky Station,Phillips Hub,Markham Hub,Mitchell Silo"
+ },
+ {
+ "name": "BD+29 3029",
+ "pos_x": -54.03125,
+ "pos_y": 37.90625,
+ "pos_z": 40.59375,
+ "stations": "Nolan Landing"
+ },
+ {
+ "name": "BD+30 4803",
+ "pos_x": -41.8125,
+ "pos_y": -95.625,
+ "pos_z": 37.0625,
+ "stations": "Wellman Mines,Gutierrez Station"
+ },
+ {
+ "name": "BD+31 2290",
+ "pos_x": 5.4375,
+ "pos_y": 81.84375,
+ "pos_z": -20.4375,
+ "stations": "Moore Terminal,Ross Hub,Caillie Depot,Budrys Dock"
+ },
+ {
+ "name": "BD+31 2373",
+ "pos_x": -1.53125,
+ "pos_y": 97.3125,
+ "pos_z": -9.15625,
+ "stations": "Beltrami Hub,Gaultier de Varennes Orbital,Lopez de Villalobos Terminal,Bellamy Port,Lubin Enterprise,Herbert Landing,Barnaby Port,Artin Station,Russell's Inheritance,Clair's Pride,Williams Terminal"
+ },
+ {
+ "name": "BD+31 434",
+ "pos_x": -51.09375,
+ "pos_y": -44.84375,
+ "pos_z": -75.125,
+ "stations": "Baudry Settlement,Lister Holdings,Platt Base"
+ },
+ {
+ "name": "BD+32 4747",
+ "pos_x": -77.75,
+ "pos_y": -43.96875,
+ "pos_z": -30.4375,
+ "stations": "Bridges City,Bluford Colony,Benford Vision"
+ },
+ {
+ "name": "BD+33 1931",
+ "pos_x": 15.0625,
+ "pos_y": 86.5,
+ "pos_z": -62.8125,
+ "stations": "Kennicott Terminal,Crown Hub"
+ },
+ {
+ "name": "BD+33 2071",
+ "pos_x": 10.59375,
+ "pos_y": 109.34375,
+ "pos_z": -46.03125,
+ "stations": "H. G. Wells Survey,Cartan Penitentiary,Barcelo Mines,al-Khowarizmi Mine"
+ },
+ {
+ "name": "BD+33 256",
+ "pos_x": -23.15625,
+ "pos_y": -69.71875,
+ "pos_z": -31.59375,
+ "stations": "Barmin Laboratory,Lunan Dock,Hui Ring,Weil City,Stasheff Vision"
+ },
+ {
+ "name": "BD+33 2990",
+ "pos_x": -64.46875,
+ "pos_y": 35.09375,
+ "pos_z": 38.3125,
+ "stations": "Bulmer Dock,Swanwick Barracks,Bear Base"
+ },
+ {
+ "name": "BD+33 3582",
+ "pos_x": -64.125,
+ "pos_y": 5.09375,
+ "pos_z": 24.6875,
+ "stations": "Diamond Port,Darboux Station,Baker Port,Fraas Settlement,Lerman Prospect,Dedekind Exchange,Lehtonen Dock,Palmer Market,Giles Dock,Payne Port,Yang Vista,Shunn Point"
+ },
+ {
+ "name": "BD+33 801",
+ "pos_x": -31.3125,
+ "pos_y": -25.03125,
+ "pos_z": -107.875,
+ "stations": "Khan Installation,Apt Plant"
+ },
+ {
+ "name": "BD+34 1378",
+ "pos_x": 0.1875,
+ "pos_y": 19.8125,
+ "pos_z": -93.90625,
+ "stations": "Scortia Port,Malaspina Arsenal,Vance Terminal,Oramus Lab"
+ },
+ {
+ "name": "BD+34 1740",
+ "pos_x": 10.84375,
+ "pos_y": 47.65625,
+ "pos_z": -82,
+ "stations": "Jordan Stop"
+ },
+ {
+ "name": "BD+35 3145",
+ "pos_x": -55.84375,
+ "pos_y": 28.25,
+ "pos_z": 29.0625,
+ "stations": "Metcalf Vision"
+ },
+ {
+ "name": "BD+35 4893",
+ "pos_x": -96.34375,
+ "pos_y": -35.5625,
+ "pos_z": -13.53125,
+ "stations": "Pascal Station,Wakata Terminal"
+ },
+ {
+ "name": "BD+35 647",
+ "pos_x": -50.0625,
+ "pos_y": -35.6875,
+ "pos_z": -98.53125,
+ "stations": "Martinez Platform,Hoffman Refinery"
+ },
+ {
+ "name": "BD+37 2416",
+ "pos_x": -22.96875,
+ "pos_y": 103.25,
+ "pos_z": 3.59375,
+ "stations": "Virchow Installation"
+ },
+ {
+ "name": "BD+37 2625",
+ "pos_x": -55,
+ "pos_y": 100.28125,
+ "pos_z": 32.03125,
+ "stations": "Roberts City,Laird Hub,Smith Installation,Cayley Depot,Mitchell Dock,Swift Market,Farmer City,Cooper Orbital,Lunan Plant"
+ },
+ {
+ "name": "BD+38 2037",
+ "pos_x": 6.59375,
+ "pos_y": 74.8125,
+ "pos_z": -69.15625,
+ "stations": "Crown Dock,Schilling Works"
+ },
+ {
+ "name": "BD+38 3327",
+ "pos_x": -89.0625,
+ "pos_y": 27.90625,
+ "pos_z": 35,
+ "stations": "Lynn Base"
+ },
+ {
+ "name": "BD+39 3048",
+ "pos_x": -52.15625,
+ "pos_y": 48.03125,
+ "pos_z": 26.78125,
+ "stations": "MacDonald Colony,Froude Settlement"
+ },
+ {
+ "name": "BD+39 876",
+ "pos_x": -42.40625,
+ "pos_y": -20.0625,
+ "pos_z": -100.0625,
+ "stations": "Rasmussen Orbital,Collins Port,Fanning Dock,Kier Orbital,Mitropoulos Dock,Serebrov Silo,Scalzi Dock,Maine Enterprise,Haldeman Orbital"
+ },
+ {
+ "name": "BD+40 2208",
+ "pos_x": 2.6875,
+ "pos_y": 53.53125,
+ "pos_z": -51.21875,
+ "stations": "Rashid Refinery,Watson Colony,Oswald Gateway"
+ },
+ {
+ "name": "BD+40 2903",
+ "pos_x": -39,
+ "pos_y": 58.625,
+ "pos_z": 19.15625,
+ "stations": "Hahn Dock,Wilson Survey,Volterra Installation"
+ },
+ {
+ "name": "BD+41 1865",
+ "pos_x": 0.28125,
+ "pos_y": 29.25,
+ "pos_z": -37.53125,
+ "stations": "Cori City,Porsche Bastion"
+ },
+ {
+ "name": "BD+41 2026",
+ "pos_x": 1.78125,
+ "pos_y": 86.375,
+ "pos_z": -67.3125,
+ "stations": "Arnason Co-operative,Heyerdahl Bastion,Darboux Dock,McMahon Port"
+ },
+ {
+ "name": "BD+41 3013",
+ "pos_x": -94.0625,
+ "pos_y": 45.125,
+ "pos_z": 36.40625,
+ "stations": "Swift Colony,Bachman Port,Linenger Installation,Shukor Base"
+ },
+ {
+ "name": "BD+42 3917",
+ "pos_x": -76.53125,
+ "pos_y": -2.21875,
+ "pos_z": 7.75,
+ "stations": "Rominger Orbital,Dutton Terminal,Higginbotham Works"
+ },
+ {
+ "name": "BD+43 2989",
+ "pos_x": -104.5,
+ "pos_y": 44.34375,
+ "pos_z": 33.3125,
+ "stations": "Levi-Strauss Hub,Crown Dock,Schirra Station,Read Hub,Melvill Orbital,McNair Orbital,Newton Orbital,Leckie Prospect,Delany Installation,Harper Landing,Zindell's Claim,Ogden Point,Khrenov Horizons"
+ },
+ {
+ "name": "BD+43 866",
+ "pos_x": -45.25,
+ "pos_y": -12.78125,
+ "pos_z": -101.15625,
+ "stations": "Navigator Dock,Stewart Point,Sargent Enterprise,Morrison Port,Smith Orbital,Sellings Terminal,Rasch Terminal,McQuay Port,Abel Port,Aldiss Vista,Nikitin Palace,Collins Penal colony"
+ },
+ {
+ "name": "BD+44 1710",
+ "pos_x": -4.71875,
+ "pos_y": 44.09375,
+ "pos_z": -71.1875,
+ "stations": "Garratt Settlement,Lazarev Settlement"
+ },
+ {
+ "name": "BD+44 2250",
+ "pos_x": -27.03125,
+ "pos_y": 96.53125,
+ "pos_z": -11.875,
+ "stations": "Rose Refinery,Efremov Refinery"
+ },
+ {
+ "name": "BD+44 4389",
+ "pos_x": -61.71875,
+ "pos_y": -17.03125,
+ "pos_z": -19.21875,
+ "stations": "Vinogradov City,Shiras Installation,Godwin Prospect"
+ },
+ {
+ "name": "BD+45 2765",
+ "pos_x": -106.5625,
+ "pos_y": 40.40625,
+ "pos_z": 27.71875,
+ "stations": "Musa al-Khwarizmi Platform,Adamson Terminal,Weiss Depot"
+ },
+ {
+ "name": "BD+46 2014",
+ "pos_x": -56.28125,
+ "pos_y": 94.21875,
+ "pos_z": 13.3125,
+ "stations": "Margulies Dock,Friend Hub,Hillary Dock,Stross Dock,Simak Terminal,Ogden Station,Willis Dock,O'Donnell Holdings,Naddoddur Laboratory,Eisenstein Terminal,Martin Port,Vespucci Hub,Altshuller Hub"
+ },
+ {
+ "name": "BD+47 1236",
+ "pos_x": -22.90625,
+ "pos_y": 20.65625,
+ "pos_z": -88.09375,
+ "stations": "Dana Relay,Spedding Point"
+ },
+ {
+ "name": "BD+47 2112",
+ "pos_x": -14.78125,
+ "pos_y": 33.46875,
+ "pos_z": -0.40625,
+ "stations": "Orsini Mining Platform,Hieb Settlement"
+ },
+ {
+ "name": "BD+47 2267",
+ "pos_x": -72.78125,
+ "pos_y": 90.65625,
+ "pos_z": 19.9375,
+ "stations": "Lawson Station,Archambault Ring,Herrington Terminal,Melroy Hub,Griffiths Enterprise"
+ },
+ {
+ "name": "BD+47 2391",
+ "pos_x": -75.28125,
+ "pos_y": 64.5625,
+ "pos_z": 21.90625,
+ "stations": "Levi-Strauss Station,Galvani Terminal,Barratt Depot,Williams Beacon,Zhen Hub,vo Gateway,Thomas Port,Leeuwenhoek Gateway,Ivins Point,Grabe City,Fernandez Hub,Reynolds Dock,Springer Legacy,McKee Laboratory"
+ },
+ {
+ "name": "BD+47 2936",
+ "pos_x": -118.96875,
+ "pos_y": 22.5,
+ "pos_z": 16.5625,
+ "stations": "Oppenheimer Mine,Bondar Terminal"
+ },
+ {
+ "name": "BD+48 1845B",
+ "pos_x": -11.4375,
+ "pos_y": 76.4375,
+ "pos_z": -55.625,
+ "stations": "Gardner Silo,Tennyson d'Eyncourt Point,Kozin Beacon"
+ },
+ {
+ "name": "BD+48 738",
+ "pos_x": -93.53125,
+ "pos_y": -24.46875,
+ "pos_z": -114.71875,
+ "stations": "Fanning's Inheritance,Linnaeus Enterprise,Atwater Station,Morin Terminal,Carleson Depot"
+ },
+ {
+ "name": "BD+49 1280",
+ "pos_x": -19.84375,
+ "pos_y": 4.625,
+ "pos_z": -49.15625,
+ "stations": "Hale City,Higginbotham Dock,Laplace Orbital,Archimedes City,Stewart Terminal,Tull Gateway,Sharipov Dock,Kovalevsky Orbital,Vishweswarayya Silo,Gold Vista,Nikolayev Port,Nakaya Hub,Gantt Installation"
+ },
+ {
+ "name": "BD+49 3937",
+ "pos_x": -93.15625,
+ "pos_y": -13.09375,
+ "pos_z": -22.84375,
+ "stations": "Maxwell City,Kovalevsky Orbital,Jendrassik Survey,Onufrienko Base"
+ },
+ {
+ "name": "BD+50 1864",
+ "pos_x": -24.875,
+ "pos_y": 98.5,
+ "pos_z": -37.71875,
+ "stations": "Rothman Dock,Anthony de la Roche Hub,Gardner Installation,Rowley Survey"
+ },
+ {
+ "name": "BD+52 3410",
+ "pos_x": -62.65625,
+ "pos_y": -8.0625,
+ "pos_z": -22,
+ "stations": "Payton Port,Knight Hub,Oleskiw Holdings,Locke's Folly"
+ },
+ {
+ "name": "BD+53 912",
+ "pos_x": 115.84375,
+ "pos_y": -14.28125,
+ "pos_z": 18.5625,
+ "stations": "Blaha Dock,Behnken Station,Crown Station"
+ },
+ {
+ "name": "BD+55 1142",
+ "pos_x": -26.09375,
+ "pos_y": 34.1875,
+ "pos_z": -75.21875,
+ "stations": "Humphreys Terminal,Taine Vision,Barnes Horizons,Belyanin Survey,Brillant City"
+ },
+ {
+ "name": "BD+55 1519",
+ "pos_x": -16.9375,
+ "pos_y": 44.71875,
+ "pos_z": -16.59375,
+ "stations": "Schirra Port,Arber Colony"
+ },
+ {
+ "name": "BD+55 3074",
+ "pos_x": -161.03125,
+ "pos_y": -16.75,
+ "pos_z": -81,
+ "stations": "Cabral Stop,McAllaster Works,Rodrigues Relay"
+ },
+ {
+ "name": "BD+55 570",
+ "pos_x": -56.375,
+ "pos_y": -5.96875,
+ "pos_z": -55.84375,
+ "stations": "Ray Orbital,Cartan Relay,Becquerel Keep"
+ },
+ {
+ "name": "BD+56 1773",
+ "pos_x": -69.3125,
+ "pos_y": 93.375,
+ "pos_z": -3.6875,
+ "stations": "Barjavel Dock,Bertin City,Bellamy Point,Boulle Holdings"
+ },
+ {
+ "name": "BD+57 1692",
+ "pos_x": -76.875,
+ "pos_y": 65.8125,
+ "pos_z": 4.625,
+ "stations": "Park's Pride"
+ },
+ {
+ "name": "BD+57 2595",
+ "pos_x": -110.40625,
+ "pos_y": -1.25,
+ "pos_z": -34.25,
+ "stations": "Flynn Ring,Smith Enterprise,Conklin Station,Furukawa Laboratory,Hopkins Terminal,Appel Point"
+ },
+ {
+ "name": "BD+58 1208",
+ "pos_x": -27.875,
+ "pos_y": 73.03125,
+ "pos_z": -65.90625,
+ "stations": "Carstensz Gateway,Gilliland Ring,Griffin Station,Varley Barracks,Amundsen Enterprise,Wilson Installation"
+ },
+ {
+ "name": "BD+59 1851",
+ "pos_x": 58,
+ "pos_y": -37.53125,
+ "pos_z": 86.5625,
+ "stations": "Guin Bastion,Lethem Landing,Norman Installation,Baxter Colony,Burckhardt Dock"
+ },
+ {
+ "name": "BD+60 585",
+ "pos_x": -79.28125,
+ "pos_y": 3.6875,
+ "pos_z": -86.71875,
+ "stations": "Coles Depot"
+ },
+ {
+ "name": "BD+63 1764",
+ "pos_x": -113.25,
+ "pos_y": 16.78125,
+ "pos_z": -28.34375,
+ "stations": "Narbeth Port,Hasse City,Hambly Laboratory,Nemere City,Phillifent City,Alpers Ring,Pascal Beacon,Plante Point"
+ },
+ {
+ "name": "BD+64 1452",
+ "pos_x": -98.5625,
+ "pos_y": 25.6875,
+ "pos_z": -17.40625,
+ "stations": "Sakers Mine,Cleve Point"
+ },
+ {
+ "name": "BD+65 1846",
+ "pos_x": -60.3125,
+ "pos_y": 7.125,
+ "pos_z": -25.53125,
+ "stations": "Olivas Terminal,Lander Legacy,Oswald Port,Kraepelin Enterprise,Banks Dock,Hopkins' Progress,Debye Orbital,Brunton Station,Hawley Enterprise,Thiele Hub,Adamson Terminal,Cameron Terminal,Toll Bastion,Baxter Prospect,Joule Depot,Soddy Palace"
+ },
+ {
+ "name": "BD+65 210",
+ "pos_x": -56.53125,
+ "pos_y": 5.625,
+ "pos_z": -46.65625,
+ "stations": "Stefanyshyn-Piper Port,Baraniecki Arsenal,Belyayev Gateway,Duckworth Terminal"
+ },
+ {
+ "name": "BD+65 626",
+ "pos_x": -48.34375,
+ "pos_y": 66.96875,
+ "pos_z": -85.6875,
+ "stations": "Chalker Dock,Macquorn Rankine's Inheritance"
+ },
+ {
+ "name": "BD+66 193",
+ "pos_x": -57.53125,
+ "pos_y": 8.625,
+ "pos_z": -50.21875,
+ "stations": "Ockels Vista,Goeschke Colony,Samokutyayev Dock,Kregel Terminal"
+ },
+ {
+ "name": "BD+66 696",
+ "pos_x": -52.4375,
+ "pos_y": 89.0625,
+ "pos_z": -59.53125,
+ "stations": "Fernao do Po City,Lunan Terminal,Antonio de Andrade Orbital,Fife Gateway"
+ },
+ {
+ "name": "BD+67 1409",
+ "pos_x": -89.4375,
+ "pos_y": 16.0625,
+ "pos_z": -30.25,
+ "stations": "Le Guin Terminal,Crichton Station,Brillant Dock,Grabe Barracks"
+ },
+ {
+ "name": "BD+69 238",
+ "pos_x": -39.03125,
+ "pos_y": 14.1875,
+ "pos_z": -44.90625,
+ "stations": "Reed Plant,Rubruck Enterprise"
+ },
+ {
+ "name": "BD+69 530",
+ "pos_x": -61.21875,
+ "pos_y": 81.5,
+ "pos_z": -37.75,
+ "stations": "Leoniceno Orbital,Burbank Gateway,Hippalus Beacon,Bellamy Base,Popovich Terminal"
+ },
+ {
+ "name": "BD+70 578",
+ "pos_x": -51.9375,
+ "pos_y": 71.5,
+ "pos_z": -65.90625,
+ "stations": "Ciferri Stop,Eisele Camp"
+ },
+ {
+ "name": "BD+71 1033",
+ "pos_x": -104.125,
+ "pos_y": 33.875,
+ "pos_z": -30.9375,
+ "stations": "Vela Station"
+ },
+ {
+ "name": "BD+71 789",
+ "pos_x": -84.5625,
+ "pos_y": 65.75,
+ "pos_z": -21.25,
+ "stations": "Petaja Enterprise,Wilcutt Relay"
+ },
+ {
+ "name": "BD+72 545",
+ "pos_x": -48.375,
+ "pos_y": 61.71875,
+ "pos_z": -40.21875,
+ "stations": "Rangarajan Enterprise,Gardner Platform,Acharya Relay,Varthema Enterprise"
+ },
+ {
+ "name": "BD+73 447",
+ "pos_x": -59.8125,
+ "pos_y": 67.21875,
+ "pos_z": -70.625,
+ "stations": "Johnson City,Markov Gateway,Lee Landing,Alvares Survey,Desargues Bastion"
+ },
+ {
+ "name": "BD+74 526",
+ "pos_x": -48.65625,
+ "pos_y": 53.28125,
+ "pos_z": -29.25,
+ "stations": "Krusvar Station,Butcher Beacon,Potagos Holdings"
+ },
+ {
+ "name": "BD+75 58",
+ "pos_x": -43.5625,
+ "pos_y": 13.46875,
+ "pos_z": -30.46875,
+ "stations": "Stephenson Station,Currie Enterprise,Burnet Survey"
+ },
+ {
+ "name": "BD+77 84",
+ "pos_x": -88.125,
+ "pos_y": 32.8125,
+ "pos_z": -70.40625,
+ "stations": "Chwedyk Port"
+ },
+ {
+ "name": "BD+87 118",
+ "pos_x": -58.59375,
+ "pos_y": 40.65625,
+ "pos_z": -37.75,
+ "stations": "Magnus Point,Patrick Ring"
+ },
+ {
+ "name": "BD-00 3426",
+ "pos_x": -45.53125,
+ "pos_y": 14.59375,
+ "pos_z": 85.125,
+ "stations": "Dirac Station,Napier Base,Grijalva Observatory,Fischer Port,Flynn's Exile,Kregel Orbital,Crown Station,Patsayev Port,Atwater City,Popovich Port,Tito Horizons,Clapperton Silo,Polansky Reach"
+ },
+ {
+ "name": "BD-00 4461",
+ "pos_x": -66.15625,
+ "pos_y": -91.34375,
+ "pos_z": 15.71875,
+ "stations": "Webb Orbital,Piccard Hub,Flint Station"
+ },
+ {
+ "name": "BD-00 632",
+ "pos_x": 9.46875,
+ "pos_y": -36.34375,
+ "pos_z": -48.625,
+ "stations": "McCormick Port,Ramaswamy Ring,Grandin Ring,Singer Gateway,Lawrence City,Henslow Installation,Cook Survey"
+ },
+ {
+ "name": "BD-01 1707",
+ "pos_x": 72.875,
+ "pos_y": 14.40625,
+ "pos_z": -89.125,
+ "stations": "Behnken Terminal,Boming Gateway,Lamarck Enterprise,Porsche City,Serling Legacy,Clark City,Kubasov City,Khan Port,Gurragchaa Gateway,Bowersox Enterprise,Gardner Hub,Houtman Terminal"
+ },
+ {
+ "name": "BD-01 2518",
+ "pos_x": 125.9375,
+ "pos_y": 9.4375,
+ "pos_z": 49.53125,
+ "stations": "Watson Terminal,Papin Orbital,Levchenko Station,Andreas' Claim,Block Landing"
+ },
+ {
+ "name": "BD-01 2784",
+ "pos_x": 23.84375,
+ "pos_y": 57.78125,
+ "pos_z": 23.71875,
+ "stations": "Resnik Dock,Xiaoguan Plant,Curie Orbital,Haxel Enterprise,Tavernier Landing"
+ },
+ {
+ "name": "BD-01 3500",
+ "pos_x": -29.5625,
+ "pos_y": 3.59375,
+ "pos_z": 52.90625,
+ "stations": "Stafford's Progress,Bergerac Survey,Bosch Dock,Griffin Prospect,Liska Prospect"
+ },
+ {
+ "name": "BD-02 4304",
+ "pos_x": -30.75,
+ "pos_y": 38.15625,
+ "pos_z": 94.6875,
+ "stations": "Brandenstein Enterprise,Gell-Mann Dock,Parker Beacon,Currie Terminal,Bean Enterprise,Durrance Station,Newton Dock,Williamson Terminal,Fabian Dock,Abbott Arsenal,Tanner Installation,Simak Town"
+ },
+ {
+ "name": "BD-02 5286",
+ "pos_x": -65.5,
+ "pos_y": -40.125,
+ "pos_z": 69.09375,
+ "stations": "Thorne Terminal,Borlaug Dock,Clark City,Selye Hub,Rukavishnikov Orbital,Rennie Relay,Elion Dock,Tucker Silo,Farouk Prospect"
+ },
+ {
+ "name": "BD-04 2421",
+ "pos_x": 79.65625,
+ "pos_y": 41.65625,
+ "pos_z": -63.90625,
+ "stations": "Haipeng Ring,Kuhn Terminal,Phillips Ring,Foda Dock,Ericsson Penal colony,Shepherd Enterprise"
+ },
+ {
+ "name": "BD-04 4138",
+ "pos_x": -19.375,
+ "pos_y": 45.96875,
+ "pos_z": 92.625,
+ "stations": "Gardner Prospect,Shunn Dock,Siodmak Dock,MacKellar Bastion"
+ },
+ {
+ "name": "BD-04 797",
+ "pos_x": 23.09375,
+ "pos_y": -52.71875,
+ "pos_z": -71.96875,
+ "stations": "Parker Dock,Hobaugh Settlement,Vetulani Hub,Haise Settlement"
+ },
+ {
+ "name": "BD-05 2489",
+ "pos_x": 83.34375,
+ "pos_y": 33.96875,
+ "pos_z": -72.625,
+ "stations": "Converse Camp,Wheeler Hub,Pelliot Hub"
+ },
+ {
+ "name": "BD-05 2603",
+ "pos_x": 73.21875,
+ "pos_y": 35.4375,
+ "pos_z": -56.03125,
+ "stations": "Ford Ring,Soddy Relay"
+ },
+ {
+ "name": "BD-05 2778",
+ "pos_x": 56.21875,
+ "pos_y": 37.84375,
+ "pos_z": -34.78125,
+ "stations": "Ramon Settlement,Al Saud Retreat"
+ },
+ {
+ "name": "BD-05 5480",
+ "pos_x": -53.71875,
+ "pos_y": -47.9375,
+ "pos_z": 51.1875,
+ "stations": "Cauchy Bastion,Richards Beacon,Taylor Orbital"
+ },
+ {
+ "name": "BD-05 948",
+ "pos_x": 35.5,
+ "pos_y": -63.90625,
+ "pos_z": -93.90625,
+ "stations": "Derleth Platform,Martinez Orbital"
+ },
+ {
+ "name": "BD-06 2528",
+ "pos_x": 80.4375,
+ "pos_y": 29.40625,
+ "pos_z": -68.5625,
+ "stations": "Bramah Camp"
+ },
+ {
+ "name": "BD-07 4419",
+ "pos_x": -7.96875,
+ "pos_y": 9,
+ "pos_z": 30,
+ "stations": "Laveykin Platform,Hale Hangar,Lee's Folly"
+ },
+ {
+ "name": "BD-08 2963",
+ "pos_x": 87.03125,
+ "pos_y": 79.59375,
+ "pos_z": -20.28125,
+ "stations": "O'Leary Colony,Blaylock Enterprise,Vetulani Point"
+ },
+ {
+ "name": "BD-08 535",
+ "pos_x": 5.34375,
+ "pos_y": -82,
+ "pos_z": -56.625,
+ "stations": "Wakata Beacon,Mohmand's Claim"
+ },
+ {
+ "name": "BD-09 241",
+ "pos_x": -24.03125,
+ "pos_y": -105.59375,
+ "pos_z": -29.90625,
+ "stations": "Herndon Survey,Goonan Hangar,Aikin Terminal"
+ },
+ {
+ "name": "BD-09 2535",
+ "pos_x": 84.6875,
+ "pos_y": 31.875,
+ "pos_z": -61,
+ "stations": "Bretnor Vision"
+ },
+ {
+ "name": "BD-09 4592",
+ "pos_x": -31.40625,
+ "pos_y": 19.40625,
+ "pos_z": 102.3125,
+ "stations": "Tsunenaga Hangar,Archimedes Base,Weiss Estate"
+ },
+ {
+ "name": "BD-10 4011",
+ "pos_x": 10.78125,
+ "pos_y": 40.5,
+ "pos_z": 47.125,
+ "stations": "Sinclair Port,Helmholtz Beacon,Timofeyevich Barracks"
+ },
+ {
+ "name": "BD-10 5142",
+ "pos_x": -49.09375,
+ "pos_y": -28.25,
+ "pos_z": 87.28125,
+ "stations": "Piccard Platform"
+ },
+ {
+ "name": "BD-10 5238",
+ "pos_x": -36.0625,
+ "pos_y": -25.03125,
+ "pos_z": 57.59375,
+ "stations": "Euclid Camp,Weyn Port,Heceta Hub"
+ },
+ {
+ "name": "BD-11 4280",
+ "pos_x": -17.625,
+ "pos_y": 33.84375,
+ "pos_z": 108.25,
+ "stations": "Mitchell City,Panshin Enterprise"
+ },
+ {
+ "name": "BD-11 4932",
+ "pos_x": -41.96875,
+ "pos_y": -18.65625,
+ "pos_z": 84.8125,
+ "stations": "Morukov Station,Shatalov Gateway,Helms Survey,Overmyer Enterprise,Slonczewski Plant,Davy Orbital"
+ },
+ {
+ "name": "BD-11 5484",
+ "pos_x": -54.375,
+ "pos_y": -58.40625,
+ "pos_z": 68.5625,
+ "stations": "Collins Station,Gooch Orbital,Freud Orbital,Roosa Hub,Conway Gateway,Lovelace Dock,Zahn Depot,Dampier Exchange,Fozard Settlement,Brunel Observatory"
+ },
+ {
+ "name": "BD-12 4699",
+ "pos_x": -16.34375,
+ "pos_y": 21.6875,
+ "pos_z": 84.53125,
+ "stations": "Narita Hub,Bushkov Station,Witt Station,Krusvar Installation,Gagnan Landing,Alexander Port,Scheerbart City,Wafer Depot,Davis Station,Janifer Orbital,Stevin Port,Hughes Dock,Mitchison Enterprise"
+ },
+ {
+ "name": "BD-12 5409",
+ "pos_x": -51.65625,
+ "pos_y": -29.21875,
+ "pos_z": 103.65625,
+ "stations": "Lopez de Legazpi Ring,Slade Orbital,Sleator Gateway,Otiman Enterprise"
+ },
+ {
+ "name": "BD-12 6174",
+ "pos_x": -34.84375,
+ "pos_y": -54.5625,
+ "pos_z": 32.625,
+ "stations": "Kuipers Point,Overmyer City,Sacco Installation"
+ },
+ {
+ "name": "BD-13 2439",
+ "pos_x": 56.9375,
+ "pos_y": 14,
+ "pos_z": -39.28125,
+ "stations": "Abbott Survey,Ostrander Station"
+ },
+ {
+ "name": "BD-13 6060",
+ "pos_x": -51.65625,
+ "pos_y": -80.4375,
+ "pos_z": 52.34375,
+ "stations": "Hauck Orbital,Velazquez Hangar,Aristotle Hub,Robinson Prospect"
+ },
+ {
+ "name": "BD-14 3326",
+ "pos_x": 85.84375,
+ "pos_y": 79.34375,
+ "pos_z": 8.6875,
+ "stations": "Siegel Port,Jemison's Inheritance,Robinson Station,Kettle Landing,Santos Barracks,Sleator's Folly"
+ },
+ {
+ "name": "BD-15 2506",
+ "pos_x": 97.1875,
+ "pos_y": 29.28125,
+ "pos_z": -55.53125,
+ "stations": "Kregel Colony"
+ },
+ {
+ "name": "BD-15 4230",
+ "pos_x": 6.53125,
+ "pos_y": 51.40625,
+ "pos_z": 101.46875,
+ "stations": "Treshchov Port,Read City"
+ },
+ {
+ "name": "BD-15 447",
+ "pos_x": 8,
+ "pos_y": -81.25,
+ "pos_z": -40.125,
+ "stations": "Good Enterprise,Gutierrez Port,Wilmore Landing,Banks Hub,Jordan Point"
+ },
+ {
+ "name": "BD-16 2761",
+ "pos_x": 94.15625,
+ "pos_y": 42.78125,
+ "pos_z": -37.6875,
+ "stations": "Smith Dock,d'Eyncourt Horizons"
+ },
+ {
+ "name": "BD-17 1955",
+ "pos_x": 93.28125,
+ "pos_y": -1.09375,
+ "pos_z": -71.84375,
+ "stations": "Klein Survey,Wang Gateway,Harbaugh Ring"
+ },
+ {
+ "name": "BD-17 4631",
+ "pos_x": -4.125,
+ "pos_y": 34.71875,
+ "pos_z": 113.8125,
+ "stations": "Schmidt Hub,Khrunov Hangar,Borman Oasis,Evans Terminal"
+ },
+ {
+ "name": "BD-17 5832",
+ "pos_x": -42.625,
+ "pos_y": -43.03125,
+ "pos_z": 89.53125,
+ "stations": "Byrd Station,Milnor Gateway,Shiner Station,Sturckow Hub,Volterra Station,Valdes Ring,Dupuy de Lome Survey"
+ },
+ {
+ "name": "BD-17 6081",
+ "pos_x": -48.625,
+ "pos_y": -64.71875,
+ "pos_z": 84.75,
+ "stations": "MacKellar Dock,Carpenter Enterprise,Garneau Hub,Perry Works,Pailes Port,Irwin Port,Barth Depot"
+ },
+ {
+ "name": "BD-17 6172",
+ "pos_x": -29.0625,
+ "pos_y": -41.90625,
+ "pos_z": 47.09375,
+ "stations": "Reed Orbital,Roddenberry City,Cochrane Gateway,Heck Dock,Chun Market,Loncke Terminal,Alvares Settlement,Cook Port,Deslandres Survey,Martin Depot"
+ },
+ {
+ "name": "BD-18 3106",
+ "pos_x": 48.46875,
+ "pos_y": 36.875,
+ "pos_z": 2.0625,
+ "stations": "Worden Dock,Clifford Gateway,Jones Depot,Remec Installation"
+ },
+ {
+ "name": "BD-18 394",
+ "pos_x": 6.3125,
+ "pos_y": -70.03125,
+ "pos_z": -27.53125,
+ "stations": "Vernadsky Camp,McDivitt Terminal,Moisuc Refinery,Hilmers Relay"
+ },
+ {
+ "name": "BD-19 3629A",
+ "pos_x": 54.21875,
+ "pos_y": 61.40625,
+ "pos_z": 41.8125,
+ "stations": "Friesner Port,Gaultier de Varennes Dock,Rawat Dock,Reed Dock,Rowley's Claim,Staden Orbital,Mawson Vista,Phillpotts' Inheritance,Walheim Prospect,Henslow Palace,Cantor Terminal,Rose City,Payne's Progress,Peral Vision,Shipton City,Serre Dock"
+ },
+ {
+ "name": "BD-19 402",
+ "pos_x": -104.4375,
+ "pos_y": 0.46875,
+ "pos_z": 14.625,
+ "stations": "Cavendish Station,Laval Survey,vo Settlement"
+ },
+ {
+ "name": "BD-20 2665",
+ "pos_x": 105.6875,
+ "pos_y": 27.5,
+ "pos_z": -46.8125,
+ "stations": "Curie Port,Cleave Station,Thoreau Relay,Bogdanov Laboratory"
+ },
+ {
+ "name": "BD-21 1074",
+ "pos_x": 22.4375,
+ "pos_y": -20.9375,
+ "pos_z": -24.53125,
+ "stations": "Snyder Mine"
+ },
+ {
+ "name": "BD-21 3153",
+ "pos_x": 89.96875,
+ "pos_y": 56.84375,
+ "pos_z": -1,
+ "stations": "Torricelli Station"
+ },
+ {
+ "name": "BD-21 3154",
+ "pos_x": 95.09375,
+ "pos_y": 60,
+ "pos_z": -0.9375,
+ "stations": "Howard Hub,H. G. Wells Beacon"
+ },
+ {
+ "name": "BD-21 4791",
+ "pos_x": -50.375,
+ "pos_y": -62.40625,
+ "pos_z": -65.90625,
+ "stations": "Zudov Dock,Shargin Station,Eddington Port,Herschel Terminal,Burbank Port,Leibniz Enterprise"
+ },
+ {
+ "name": "BD-22 1050",
+ "pos_x": 67.03125,
+ "pos_y": -56.625,
+ "pos_z": -70.1875,
+ "stations": "Hadfield Orbital,Barratt Port,Fernao do Po Relay,Harrison Observatory"
+ },
+ {
+ "name": "BD-22 286",
+ "pos_x": 4.1875,
+ "pos_y": -92.125,
+ "pos_z": -21.90625,
+ "stations": "Ampere Port,Jones Vision,Ford Refinery"
+ },
+ {
+ "name": "BD-22 3573",
+ "pos_x": 68.0625,
+ "pos_y": 75.3125,
+ "pos_z": 63.375,
+ "stations": "Bohm Terminal,Forrester Station,Khayyam Orbital,Planck Orbital,Teng Barracks,Jemison Beacon"
+ },
+ {
+ "name": "BD-22 5522",
+ "pos_x": -37.65625,
+ "pos_y": -63.4375,
+ "pos_z": 82.25,
+ "stations": "Dedekind Station,Maybury Survey,Galois Enterprise"
+ },
+ {
+ "name": "Bean",
+ "pos_x": -83.6875,
+ "pos_y": -41.8125,
+ "pos_z": -100.34375,
+ "stations": "Rocklynne Dock,Bruce Dock"
+ },
+ {
+ "name": "Bean Nighe",
+ "pos_x": 100.46875,
+ "pos_y": -7,
+ "pos_z": 5.6875,
+ "stations": "Krikalev Terminal,Chang-Diaz Station,Carter Park"
+ },
+ {
+ "name": "Beara",
+ "pos_x": 97.09375,
+ "pos_y": -238.78125,
+ "pos_z": 63.46875,
+ "stations": "Fischer Dock,Wylie Landing,Card Survey"
+ },
+ {
+ "name": "Beargk",
+ "pos_x": 31.71875,
+ "pos_y": -91.75,
+ "pos_z": 135.28125,
+ "stations": "Banno Orbital,Baldwin Hub,Mattingly Terminal,Hubble Settlement"
+ },
+ {
+ "name": "Beatis",
+ "pos_x": 77.9375,
+ "pos_y": -47.0625,
+ "pos_z": -12.59375,
+ "stations": "Neff Hub,Griffith Port,Reed Ring,Nelder Station,Vlamingh Hub,Fiennes City,Harper Orbital"
+ },
+ {
+ "name": "Bedaho",
+ "pos_x": 79.34375,
+ "pos_y": -21.09375,
+ "pos_z": -7.15625,
+ "stations": "Peters Terminal,Sharipov Hub,Morin Legacy,Salam Enterprise"
+ },
+ {
+ "name": "Bedaramojas",
+ "pos_x": -16.09375,
+ "pos_y": -107.9375,
+ "pos_z": 118.15625,
+ "stations": "Virts Terminal,Carlisle Landing,Frimout Hub"
+ },
+ {
+ "name": "Bedari",
+ "pos_x": -4.625,
+ "pos_y": -39.59375,
+ "pos_z": 140.6875,
+ "stations": "Russell Beacon"
+ },
+ {
+ "name": "Bedia",
+ "pos_x": 66.6875,
+ "pos_y": -39.09375,
+ "pos_z": -74.9375,
+ "stations": "Bhabha Terminal,Voss Enterprise,Drexler Dock,Remek Dock"
+ },
+ {
+ "name": "Bediae",
+ "pos_x": -55.625,
+ "pos_y": -0.46875,
+ "pos_z": -128.1875,
+ "stations": "Otiman Dock"
+ },
+ {
+ "name": "Bediangu",
+ "pos_x": 150.71875,
+ "pos_y": -98.78125,
+ "pos_z": 89.1875,
+ "stations": "Kirby Port,Gent Orbital,Fox Installation"
+ },
+ {
+ "name": "Bedimo",
+ "pos_x": -141.46875,
+ "pos_y": -27.9375,
+ "pos_z": -67.9375,
+ "stations": "Hewish Outpost"
+ },
+ {
+ "name": "Beditjari",
+ "pos_x": -6.53125,
+ "pos_y": -60.9375,
+ "pos_z": 104.34375,
+ "stations": "Graham City,Zubrin Terminal,Jones Laboratory,Abel Vision,Humphreys Vision,Schweinfurth Bastion"
+ },
+ {
+ "name": "Beetrix",
+ "pos_x": -31.90625,
+ "pos_y": -32.03125,
+ "pos_z": -74.40625,
+ "stations": "Ostwald Terminal,Hertz Market,Al-Jazari Port,Hinz Barracks,Filter Landing,Jones Port,Sadi Carnot City"
+ },
+ {
+ "name": "Begoci",
+ "pos_x": 56.53125,
+ "pos_y": -18.90625,
+ "pos_z": -164.46875,
+ "stations": "McMahon Relay"
+ },
+ {
+ "name": "Begonda",
+ "pos_x": 55.84375,
+ "pos_y": 99.21875,
+ "pos_z": -120.25,
+ "stations": "Starzl Survey"
+ },
+ {
+ "name": "Begovans",
+ "pos_x": -61.75,
+ "pos_y": 19.75,
+ "pos_z": 69.53125,
+ "stations": "Thomson Platform,Bohm City"
+ },
+ {
+ "name": "Bei Dou Sector DL-Y d76",
+ "pos_x": -37.6875,
+ "pos_y": 70.09375,
+ "pos_z": -14.1875,
+ "stations": "Detention Ship Beta"
+ },
+ {
+ "name": "Beid",
+ "pos_x": 32.1875,
+ "pos_y": -74.625,
+ "pos_z": -90.625,
+ "stations": "Ising Point"
+ },
+ {
+ "name": "Beimech",
+ "pos_x": 49.1875,
+ "pos_y": 14.875,
+ "pos_z": 71,
+ "stations": "Al-Khalili Gateway,Bresnik Terminal,Gamow Port,Aubakirov Port,MacLean Enterprise,Bayliss Ring,Hackworth Orbital,Fontana's Inheritance,Baker Base,Hughes-Fulford Gateway,Melvill Terminal,Burstein Installation,Carr Vista,Narbeth Penal colony"
+ },
+ {
+ "name": "Beiwe",
+ "pos_x": 28.90625,
+ "pos_y": -47,
+ "pos_z": -13.625,
+ "stations": "Soddy Ring"
+ },
+ {
+ "name": "Bekenam",
+ "pos_x": -101.21875,
+ "pos_y": -45.25,
+ "pos_z": 32.9375,
+ "stations": "Bertin Landing,Crown Mine,Yurchikhin Outpost"
+ },
+ {
+ "name": "Beker",
+ "pos_x": 134.59375,
+ "pos_y": -110.375,
+ "pos_z": 46.125,
+ "stations": "Maupertuis Dock,Shajn Orbital,Fiorilla Terminal,Eggleton Orbital,Pond Hub,Qureshi Station,Wild Terminal,Paulo da Gama Port,Arai Hub,Curtiss Hub,Bradshaw Works,Wigura Hub,Fox Refinery,Simak's Pride,Syromyatnikov Vista"
+ },
+ {
+ "name": "Beket",
+ "pos_x": -61.34375,
+ "pos_y": 141.5,
+ "pos_z": 36.03125,
+ "stations": "Wafer Asylum"
+ },
+ {
+ "name": "Bela",
+ "pos_x": -26.375,
+ "pos_y": 63.84375,
+ "pos_z": -137.0625,
+ "stations": "Diesel Works"
+ },
+ {
+ "name": "Bela Oh",
+ "pos_x": 157.25,
+ "pos_y": -112.71875,
+ "pos_z": 35.90625,
+ "stations": "Armero Platform,Fairey Mines,Plait Horizons,Clifford's Claim,Narita Terminal"
+ },
+ {
+ "name": "Bela Wena",
+ "pos_x": -13.46875,
+ "pos_y": -182.1875,
+ "pos_z": 81.375,
+ "stations": "Arai's Pride,Rabinowitz Survey,Burgess Point,McKee Depot"
+ },
+ {
+ "name": "Belaako",
+ "pos_x": 63.21875,
+ "pos_y": 47.375,
+ "pos_z": 140.09375,
+ "stations": "Lamarck Station,Al-Farabi Station,Duke Dock,Selye's Progress"
+ },
+ {
+ "name": "Belaba",
+ "pos_x": 85.40625,
+ "pos_y": -89.375,
+ "pos_z": 60.15625,
+ "stations": "Barr Port"
+ },
+ {
+ "name": "Belabos",
+ "pos_x": 109.78125,
+ "pos_y": -83.0625,
+ "pos_z": -61.03125,
+ "stations": "Dunyach Depot,Balog Colony,Dumont Oasis"
+ },
+ {
+ "name": "Belacabunda",
+ "pos_x": 137.90625,
+ "pos_y": 43.4375,
+ "pos_z": 76.53125,
+ "stations": "Morgan Orbital"
+ },
+ {
+ "name": "Belacajan",
+ "pos_x": -75.59375,
+ "pos_y": -99.71875,
+ "pos_z": 17.8125,
+ "stations": "Barry Outpost,Crown Base,Viehbock Prospect"
+ },
+ {
+ "name": "Belach",
+ "pos_x": 10.75,
+ "pos_y": -56.375,
+ "pos_z": -60.53125,
+ "stations": "Ross Colony"
+ },
+ {
+ "name": "Belachina",
+ "pos_x": 37.3125,
+ "pos_y": -24.15625,
+ "pos_z": -159.15625,
+ "stations": "Ibold Colony,Scott Stop"
+ },
+ {
+ "name": "Belae",
+ "pos_x": -80.5625,
+ "pos_y": -98.96875,
+ "pos_z": -80.65625,
+ "stations": "Thagard Gateway"
+ },
+ {
+ "name": "Belagro",
+ "pos_x": 74.03125,
+ "pos_y": -32.4375,
+ "pos_z": 47.25,
+ "stations": "Leclerc Installation,Hermaszewski Station"
+ },
+ {
+ "name": "Belalans",
+ "pos_x": 81.625,
+ "pos_y": -94.875,
+ "pos_z": -58.5625,
+ "stations": "Powers Hub,Mandel Dock,Boscovich Ring,Fairey Vision,Ban Hub,Luu Dock,Janszoon Depot,Shoemaker City"
+ },
+ {
+ "name": "Belalis",
+ "pos_x": -83.84375,
+ "pos_y": 78.25,
+ "pos_z": -86.375,
+ "stations": "Bennett Point,Bunsen Vision"
+ },
+ {
+ "name": "Belanit",
+ "pos_x": 58.09375,
+ "pos_y": 3.53125,
+ "pos_z": 63.9375,
+ "stations": "Pavlov Dock,Bella Survey"
+ },
+ {
+ "name": "Belarsh",
+ "pos_x": -83.625,
+ "pos_y": -39.375,
+ "pos_z": -3.03125,
+ "stations": "Trevithick Port"
+ },
+ {
+ "name": "Belarsuk",
+ "pos_x": 48.9375,
+ "pos_y": -20.78125,
+ "pos_z": 37.375,
+ "stations": "Kurland Hub,Vaez de Torres Enterprise,Walker Arsenal"
+ },
+ {
+ "name": "Belata",
+ "pos_x": 138.25,
+ "pos_y": -46.875,
+ "pos_z": -10.6875,
+ "stations": "Narlikar Orbital,Xuesen Ring"
+ },
+ {
+ "name": "Belatus",
+ "pos_x": 29.96875,
+ "pos_y": -212.0625,
+ "pos_z": -26.25,
+ "stations": "Millosevich Colony"
+ },
+ {
+ "name": "Beldarkri",
+ "pos_x": -47.25,
+ "pos_y": -43.40625,
+ "pos_z": -53.5625,
+ "stations": "Onez,Johan,Vonarburg City,Crown's Claim,Lerner Relay"
+ },
+ {
+ "name": "Beleda",
+ "pos_x": -158.90625,
+ "pos_y": -13.28125,
+ "pos_z": 56.0625,
+ "stations": "Dana Terminal,Coulomb Landing,Brongniart Base"
+ },
+ {
+ "name": "Beledos",
+ "pos_x": 95.1875,
+ "pos_y": 24.5625,
+ "pos_z": -49,
+ "stations": "Kaleri Dock,Humboldt Hub,Payne Depot"
+ },
+ {
+ "name": "Belenja",
+ "pos_x": -8.53125,
+ "pos_y": -126.78125,
+ "pos_z": 21.34375,
+ "stations": "Kuttner Hub,Couper Orbital,Danforth Hub"
+ },
+ {
+ "name": "Belenses",
+ "pos_x": 88.59375,
+ "pos_y": -33.125,
+ "pos_z": -52.75,
+ "stations": "Babcock Holdings,Shepard Dock,Sturckow Landing"
+ },
+ {
+ "name": "Belevi",
+ "pos_x": -121.40625,
+ "pos_y": -116.625,
+ "pos_z": 57.09375,
+ "stations": "Hubble Landing"
+ },
+ {
+ "name": "Belevnis",
+ "pos_x": -136.03125,
+ "pos_y": 88.4375,
+ "pos_z": -28.78125,
+ "stations": "Szameit Landing,Vasquez de Coronado Hub,Kozin Depot"
+ },
+ {
+ "name": "Belgae",
+ "pos_x": 36.53125,
+ "pos_y": -43.875,
+ "pos_z": -111.9375,
+ "stations": "Pennington Vision"
+ },
+ {
+ "name": "Belgeth",
+ "pos_x": -145.96875,
+ "pos_y": -94.90625,
+ "pos_z": 2.5625,
+ "stations": "Rowley City,Barth City,Younghusband City,Turzillo Depot,Warner Beacon"
+ },
+ {
+ "name": "Belgitan",
+ "pos_x": 156.40625,
+ "pos_y": 11.4375,
+ "pos_z": 65.90625,
+ "stations": "Evans Relay,Parazynski Orbital,Copernicus Colony,Polansky Terminal,Banach Keep"
+ },
+ {
+ "name": "Belgovara",
+ "pos_x": 79.375,
+ "pos_y": -109.59375,
+ "pos_z": -85.625,
+ "stations": "McKean Hub,Russell Gateway,Kidinnu Legacy"
+ },
+ {
+ "name": "Belianses",
+ "pos_x": 82.28125,
+ "pos_y": -1.3125,
+ "pos_z": 46.09375,
+ "stations": "Archer Settlement,Mallory's Pride"
+ },
+ {
+ "name": "Beliates",
+ "pos_x": 97.875,
+ "pos_y": -179.3125,
+ "pos_z": 138.65625,
+ "stations": "Boyer Port,Lockyer Oasis,Borrelly's Folly,Lukyanenko Silo"
+ },
+ {
+ "name": "Belie",
+ "pos_x": 16.4375,
+ "pos_y": 134.3125,
+ "pos_z": -23,
+ "stations": "Rosenberg Dock"
+ },
+ {
+ "name": "Beligamba",
+ "pos_x": 137.875,
+ "pos_y": 45.71875,
+ "pos_z": -39.59375,
+ "stations": "Gibson's Progress"
+ },
+ {
+ "name": "Belinara",
+ "pos_x": -79.34375,
+ "pos_y": 22.28125,
+ "pos_z": 36.625,
+ "stations": "Efremov City,Hopkinson Orbital,Hume Depot,Cleve Settlement"
+ },
+ {
+ "name": "Belingamal",
+ "pos_x": -89.28125,
+ "pos_y": 19.6875,
+ "pos_z": -118.4375,
+ "stations": "Shumil Platform,Phillips Enterprise,Burstein's Progress"
+ },
+ {
+ "name": "Belinichs",
+ "pos_x": 194.59375,
+ "pos_y": -171.75,
+ "pos_z": 56.34375,
+ "stations": "Bauman Terminal,Ashman Penitentiary"
+ },
+ {
+ "name": "Belinjaba",
+ "pos_x": 160.25,
+ "pos_y": -153.9375,
+ "pos_z": 147.125,
+ "stations": "Martiniere Point,Brothers Horizons"
+ },
+ {
+ "name": "Belis",
+ "pos_x": -148.75,
+ "pos_y": -21.6875,
+ "pos_z": -39.6875,
+ "stations": "Tombaugh Platform,Stirling Colony,Piper Landing,Bachman's Claim,Frobisher Bastion"
+ },
+ {
+ "name": "Belisama",
+ "pos_x": 103.15625,
+ "pos_y": -157.96875,
+ "pos_z": -41,
+ "stations": "Leonard Landing,Sakers Base,Curtis Mines"
+ },
+ {
+ "name": "Belispel",
+ "pos_x": -120.59375,
+ "pos_y": -1.5,
+ "pos_z": -57.875,
+ "stations": "Gardner Dock,Narita Terminal,Delany Dock,Apt's Progress,Tiedemann Enterprise"
+ },
+ {
+ "name": "Beliu An",
+ "pos_x": 107.53125,
+ "pos_y": 37.53125,
+ "pos_z": -89.6875,
+ "stations": "Khan City"
+ },
+ {
+ "name": "Beliukis",
+ "pos_x": 102.03125,
+ "pos_y": 25.21875,
+ "pos_z": -119.09375,
+ "stations": "Conti Orbital,Alvarez de Pineda Settlement,Sauma City,McArthur Silo,McDaniel Vision"
+ },
+ {
+ "name": "Bella",
+ "pos_x": -40.46875,
+ "pos_y": 40.15625,
+ "pos_z": -114.71875,
+ "stations": "Bernoulli Port,Crumey Point"
+ },
+ {
+ "name": "Bellapa",
+ "pos_x": 43.53125,
+ "pos_y": -4.21875,
+ "pos_z": -87.21875,
+ "stations": "Budarin Terminal"
+ },
+ {
+ "name": "Bellaung",
+ "pos_x": 181.5,
+ "pos_y": -136.125,
+ "pos_z": -2.1875,
+ "stations": "Seamans Hub,Eggen Landing,Koishikawa Orbital,Galiano Refinery,Spurzem Terminal"
+ },
+ {
+ "name": "Bellintani",
+ "pos_x": 149.65625,
+ "pos_y": 51.875,
+ "pos_z": 20.5625,
+ "stations": "Kneale Dock,Filipchenko Base"
+ },
+ {
+ "name": "Bellite",
+ "pos_x": -79.71875,
+ "pos_y": -35.53125,
+ "pos_z": -95.9375,
+ "stations": "Molina Point,Parmitano Station"
+ },
+ {
+ "name": "Belliya",
+ "pos_x": -6.21875,
+ "pos_y": -53.6875,
+ "pos_z": 108.1875,
+ "stations": "Haarsma Ring,Miletus Hub,Carrasco Orbital,Binder Gateway,McAllaster Terminal,Janszoon Hub,Szentmartony Orbital,Benyovszky Station,Christopher Refinery,Dalmas City,Serrao's Claim,Wnuk-Lipinski Settlement,Wollheim Settlement,Nagata Keep"
+ },
+ {
+ "name": "Belobog",
+ "pos_x": -13.34375,
+ "pos_y": 53.78125,
+ "pos_z": 12.5625,
+ "stations": "Kingsbury Ring,al-Haytham City,Barreiros Keep"
+ },
+ {
+ "name": "Belu",
+ "pos_x": -30.3125,
+ "pos_y": 13.3125,
+ "pos_z": 59.34375,
+ "stations": "Covey Gateway,Hennepin Arsenal,Slusser Lab"
+ },
+ {
+ "name": "Belun",
+ "pos_x": 157.03125,
+ "pos_y": -32.6875,
+ "pos_z": -31.75,
+ "stations": "Jeschke Platform"
+ },
+ {
+ "name": "Belung",
+ "pos_x": 140.21875,
+ "pos_y": -209.5625,
+ "pos_z": -0.46875,
+ "stations": "Houten-Groeneveld Mines,Albitzky Stop"
+ },
+ {
+ "name": "Bembarriosk",
+ "pos_x": 59.03125,
+ "pos_y": -13.3125,
+ "pos_z": 10.59375,
+ "stations": "Arrhenius Point,Kuipers Refinery"
+ },
+ {
+ "name": "Bemberi",
+ "pos_x": 183.25,
+ "pos_y": -157.03125,
+ "pos_z": 39.96875,
+ "stations": "Furukawa Orbital,Maitz Landing"
+ },
+ {
+ "name": "Bembo",
+ "pos_x": 43.78125,
+ "pos_y": -180.3125,
+ "pos_z": -34.53125,
+ "stations": "Pons Orbital,Arrhenius Landing,Terry Hub"
+ },
+ {
+ "name": "Ben Sakha",
+ "pos_x": 138.40625,
+ "pos_y": 44.4375,
+ "pos_z": -52.625,
+ "stations": "Holden Beacon"
+ },
+ {
+ "name": "Bena",
+ "pos_x": 99.34375,
+ "pos_y": -183.8125,
+ "pos_z": 60.375,
+ "stations": "Piazzi Dock"
+ },
+ {
+ "name": "Bena Luluwa",
+ "pos_x": 154.5,
+ "pos_y": -137.1875,
+ "pos_z": 100.21875,
+ "stations": "Coye Mine"
+ },
+ {
+ "name": "Bena Xi",
+ "pos_x": -113.3125,
+ "pos_y": -42.40625,
+ "pos_z": -92.09375,
+ "stations": "Read Station"
+ },
+ {
+ "name": "Benada",
+ "pos_x": -141.4375,
+ "pos_y": 18,
+ "pos_z": -0.375,
+ "stations": "VanderMeer Colony,Card Colony,Waldrop Barracks,Hinz Survey"
+ },
+ {
+ "name": "Benambo",
+ "pos_x": 128.125,
+ "pos_y": -72.90625,
+ "pos_z": 1,
+ "stations": "Xin Orbital,Alden Terminal,Rechtin City,Burkin Landing,Alexander Hub"
+ },
+ {
+ "name": "Benanekpeno",
+ "pos_x": -101.21875,
+ "pos_y": 65.53125,
+ "pos_z": -67.1875,
+ "stations": "Kummer Platform,Kummer Outpost"
+ },
+ {
+ "name": "Benapem",
+ "pos_x": 151.96875,
+ "pos_y": 15.625,
+ "pos_z": 33.53125,
+ "stations": "Shepherd Ring,Velazquez Gateway,Nusslein-Volhard Orbital,Naddoddur Enterprise,Mohun Bastion"
+ },
+ {
+ "name": "Benapus",
+ "pos_x": -22.6875,
+ "pos_y": 20.28125,
+ "pos_z": -121.1875,
+ "stations": "Walker City,Burgess Terminal,Kennan Orbital,Jeschke Hub,Fowler City,Phillips Hub,Heck Orbital,Beregovoi Arsenal,Morgan Dock,Soto Port,Zoline's Claim,Jemison Prospect,Paulo da Gama Port,Robinson Port"
+ },
+ {
+ "name": "Benates",
+ "pos_x": -45.15625,
+ "pos_y": -50.65625,
+ "pos_z": 35.28125,
+ "stations": "Roberts Hangar"
+ },
+ {
+ "name": "Bende",
+ "pos_x": 131.0625,
+ "pos_y": -240.875,
+ "pos_z": 74.6875,
+ "stations": "Somayaji Station,Wafa Enterprise,Tuttle Platform,Oberth Vision"
+ },
+ {
+ "name": "Bendi",
+ "pos_x": -82.8125,
+ "pos_y": 61,
+ "pos_z": 65.0625,
+ "stations": "Weitz Retreat"
+ },
+ {
+ "name": "Bengga",
+ "pos_x": -88.0625,
+ "pos_y": 39.84375,
+ "pos_z": -158.3125,
+ "stations": "Sheckley Platform"
+ },
+ {
+ "name": "Beni",
+ "pos_x": -62.96875,
+ "pos_y": 144.65625,
+ "pos_z": -33.375,
+ "stations": "Stevens Dock,Soukup Works,Hendel Dock"
+ },
+ {
+ "name": "Benie",
+ "pos_x": -18.28125,
+ "pos_y": -104.9375,
+ "pos_z": 141.34375,
+ "stations": "Carrington Beacon,Mori Barracks"
+ },
+ {
+ "name": "Beniena",
+ "pos_x": 101.96875,
+ "pos_y": -94.40625,
+ "pos_z": -39.84375,
+ "stations": "Vavrova Settlement,Bayer Orbital,Oterma Beacon"
+ },
+ {
+ "name": "Benoit",
+ "pos_x": 30.46875,
+ "pos_y": -22.65625,
+ "pos_z": -2.125,
+ "stations": "Vinogradov Station,Bamford City,Fairbairn Port,Corte-Real Installation"
+ },
+ {
+ "name": "Bente",
+ "pos_x": -112.5625,
+ "pos_y": 114.90625,
+ "pos_z": 86.21875,
+ "stations": "Nagata Base,Slade Settlement"
+ },
+ {
+ "name": "Bento",
+ "pos_x": 16.46875,
+ "pos_y": -31.0625,
+ "pos_z": 1.46875,
+ "stations": "Snow Moon,Dextergrad"
+ },
+ {
+ "name": "Benty",
+ "pos_x": 147.34375,
+ "pos_y": 5.90625,
+ "pos_z": 19.875,
+ "stations": "Beaumont Depot"
+ },
+ {
+ "name": "Benu",
+ "pos_x": 105.84375,
+ "pos_y": -9.96875,
+ "pos_z": -109.9375,
+ "stations": "Mawson Terminal,Sladek Station,Lethem Enterprise"
+ },
+ {
+ "name": "Benzaiten",
+ "pos_x": -9523.15625,
+ "pos_y": -881.875,
+ "pos_z": 19811.3125,
+ "stations": "Bisley Landing,Zhu Oasis"
+ },
+ {
+ "name": "Bered",
+ "pos_x": -70.25,
+ "pos_y": -13.65625,
+ "pos_z": 124.875,
+ "stations": "Horch Orbital,Aguirre Holdings"
+ },
+ {
+ "name": "Berentin",
+ "pos_x": 9.4375,
+ "pos_y": -199.375,
+ "pos_z": 104.25,
+ "stations": "Hughes Settlement,Weaver Landing,Haro Port,Shaver Depot"
+ },
+ {
+ "name": "Berindraci",
+ "pos_x": 169.03125,
+ "pos_y": -109.84375,
+ "pos_z": 61.71875,
+ "stations": "Peters Vision"
+ },
+ {
+ "name": "Berkanan",
+ "pos_x": -19.28125,
+ "pos_y": 67.5625,
+ "pos_z": 31,
+ "stations": "Amundsen Dock,Cremona Prospect,Schouten Platform,Barton Base"
+ },
+ {
+ "name": "Bero",
+ "pos_x": 26.5625,
+ "pos_y": 13.25,
+ "pos_z": -99.9375,
+ "stations": "Herndon Hub,Ingstad Port,Nagel Ring"
+ },
+ {
+ "name": "Beronii",
+ "pos_x": 58.8125,
+ "pos_y": 50.875,
+ "pos_z": 40.25,
+ "stations": "Howard Dock,Carver Survey"
+ },
+ {
+ "name": "Berrivi",
+ "pos_x": -51.34375,
+ "pos_y": 117.46875,
+ "pos_z": -32.34375,
+ "stations": "Rashid Station,Kuhn Orbital,Thirsk Port,White Bastion,Cixin Survey"
+ },
+ {
+ "name": "Berzites",
+ "pos_x": 25.53125,
+ "pos_y": 75.25,
+ "pos_z": 23.21875,
+ "stations": "Ponce de Leon Vision,Flinders Stop,Phillifent Enterprise"
+ },
+ {
+ "name": "Berzitibi",
+ "pos_x": 64.46875,
+ "pos_y": -9.28125,
+ "pos_z": 31.4375,
+ "stations": "Whitworth Gateway,Tucker Relay,Selye Colony,Patrick Hub"
+ },
+ {
+ "name": "Berzitici",
+ "pos_x": -28.96875,
+ "pos_y": 2.53125,
+ "pos_z": 97.875,
+ "stations": "Wankel Gateway"
+ },
+ {
+ "name": "Bes",
+ "pos_x": 131.71875,
+ "pos_y": -107.59375,
+ "pos_z": 46.4375,
+ "stations": "Loncke Dock,Stephenson Terminal,Scott Dock,Tevis Port,Krylov Terminal,Bunnell Depot,Dunyach Station,Simak Enterprise,Bischoff Dock,Roggeveen Port,Stuart Bastion"
+ },
+ {
+ "name": "Bese",
+ "pos_x": 70.8125,
+ "pos_y": -64.3125,
+ "pos_z": 56.5,
+ "stations": "Lewis Dock"
+ },
+ {
+ "name": "Beseriamo",
+ "pos_x": 131.71875,
+ "pos_y": -202.0625,
+ "pos_z": 0.1875,
+ "stations": "Pacheco Survey,Hartmann Hub,Murphy Enterprise"
+ },
+ {
+ "name": "Beserket",
+ "pos_x": -83.125,
+ "pos_y": -159.65625,
+ "pos_z": -16.4375,
+ "stations": "Nelson Gateway,Swanson Dock,Collins Station,Piserchia Prospect,Klein Relay"
+ },
+ {
+ "name": "Besta",
+ "pos_x": 136.3125,
+ "pos_y": -33.65625,
+ "pos_z": 84.84375,
+ "stations": "Rayhan al-Biruni's Progress,Lopez de Villalobos Prospect,McDevitt Settlement"
+ },
+ {
+ "name": "Bestakas",
+ "pos_x": -34.71875,
+ "pos_y": -10.125,
+ "pos_z": -100.59375,
+ "stations": "Norton Station,Morrison Installation"
+ },
+ {
+ "name": "Bestia",
+ "pos_x": -36.09375,
+ "pos_y": -1.46875,
+ "pos_z": -84.5625,
+ "stations": "Duffy Port,Ostrander Survey,Tognini Station,Gooch Works"
+ },
+ {
+ "name": "Bestii",
+ "pos_x": 11.84375,
+ "pos_y": -52.53125,
+ "pos_z": 55.4375,
+ "stations": "Bickel Station"
+ },
+ {
+ "name": "Beta Caeli",
+ "pos_x": 61.40625,
+ "pos_y": -60.8125,
+ "pos_z": -35.71875,
+ "stations": "Lloyd Port,Fernandes Orbital"
+ },
+ {
+ "name": "Beta Catonis",
+ "pos_x": -58.03125,
+ "pos_y": 59.5625,
+ "pos_z": -58.78125,
+ "stations": "Bent's Gambit,Oliver Beacon"
+ },
+ {
+ "name": "Beta Circini",
+ "pos_x": 62.375,
+ "pos_y": -2.71875,
+ "pos_z": 77.65625,
+ "stations": "Bernoulli City,Afanasyev Station,Sutcliffe Holdings,Liebig Station,Eschbach Bastion,Herrington Terminal,Thuot Hub,Reamy Observatory,Coke Prospect,Quimby Hub,Land Terminal,Haxel Hub,Citi Beacon,Wrangell Orbital,Lenoir Port"
+ },
+ {
+ "name": "Beta Comae Berenices",
+ "pos_x": -1.6875,
+ "pos_y": 29.65625,
+ "pos_z": 2.03125,
+ "stations": "Gresley Port,Kube-McDowell Relay"
+ },
+ {
+ "name": "Beta Gruis",
+ "pos_x": 21.90625,
+ "pos_y": -150.9375,
+ "pos_z": 89.75,
+ "stations": "Fraunhofer Survey,Boyajian Mines"
+ },
+ {
+ "name": "Beta Horologii",
+ "pos_x": 192.96875,
+ "pos_y": -218.46875,
+ "pos_z": 42.90625,
+ "stations": "Hanke-Woods Colony"
+ },
+ {
+ "name": "Beta Hydri",
+ "pos_x": 15.28125,
+ "pos_y": -15.6875,
+ "pos_z": 10.5625,
+ "stations": "Stevenson Base,Matteucci Enterprise,Edmondson High,Black Mausoleum,Lenoir Port,Rashid Vision"
+ },
+ {
+ "name": "Beta Octantis",
+ "pos_x": 96.21875,
+ "pos_y": -85,
+ "pos_z": 76.125,
+ "stations": "Bassford Hub"
+ },
+ {
+ "name": "Beta Pavonis",
+ "pos_x": 55.875,
+ "pos_y": -80.375,
+ "pos_z": 93.125,
+ "stations": "Severin Orbital"
+ },
+ {
+ "name": "Beta Pictoris",
+ "pos_x": 53.53125,
+ "pos_y": -32.125,
+ "pos_z": -11.03125,
+ "stations": "Gaiman Dock"
+ },
+ {
+ "name": "Beta Reticuli",
+ "pos_x": 71.34375,
+ "pos_y": -68.75,
+ "pos_z": 11.3125,
+ "stations": "Regiomontanus Dock,Hubble Orbital,Ayers Survey,Elbakyan Refinery"
+ },
+ {
+ "name": "Beta Sculptoris",
+ "pos_x": 4.875,
+ "pos_y": -164.53125,
+ "pos_z": 56.5625,
+ "stations": "Fan Horizons,J. G. Ballard Town,Smith Base"
+ },
+ {
+ "name": "Beta Trianguli Australis",
+ "pos_x": 24.53125,
+ "pos_y": -5.59375,
+ "pos_z": 31.5625,
+ "stations": "Lunan Hub,Vinge Gateway,White City,Veblen Vista,Hutchinson City,Lewis Hub,Sylvester Town,Hume Station,Flinders Orbital,Francisco de Almeida Hub,Mitchell's Pride,Lindbohm Dock"
+ },
+ {
+ "name": "Beta Volantis",
+ "pos_x": 101.71875,
+ "pos_y": -29.4375,
+ "pos_z": 18.71875,
+ "stations": "Niemeyer Dock,Oliver Relay,Yakovlev Ring,Adams Dock,Fowler Ring,Iwamoto Vision,Rasmussen Settlement,Brorsen Dock,Giger Ring,Wickramasinghe Vision,Jean Hub,Roberts Landing,Lorrah Works"
+ },
+ {
+ "name": "Beta-1 Tucanae",
+ "pos_x": 63.375,
+ "pos_y": -109.71875,
+ "pos_z": 46.71875,
+ "stations": "Adams Orbital,Hirayama Barracks"
+ },
+ {
+ "name": "Beta-2 Tucanae",
+ "pos_x": 79.09375,
+ "pos_y": -136.875,
+ "pos_z": 58.28125,
+ "stations": "Fieseler Reach,Mouchez Orbital,Payne Silo"
+ },
+ {
+ "name": "Beta-3 Tucani",
+ "pos_x": 32.25,
+ "pos_y": -55.1875,
+ "pos_z": 23.875,
+ "stations": "The Beach,Bakewell Platform,Burckhardt Relay"
+ },
+ {
+ "name": "Betel",
+ "pos_x": 43,
+ "pos_y": -4.0625,
+ "pos_z": -79.65625,
+ "stations": "Baker Enterprise,Gaspar de Lemos Orbital,Amphipolis,Poteidaia"
+ },
+ {
+ "name": "Beten",
+ "pos_x": 6.71875,
+ "pos_y": 23.375,
+ "pos_z": 55.96875,
+ "stations": "Balandin Port,Khrunov City,Mattingly Bastion,Curie Observatory,Bose Enterprise"
+ },
+ {
+ "name": "Betet",
+ "pos_x": -63.625,
+ "pos_y": -159.40625,
+ "pos_z": -39.125,
+ "stations": "Conti Terminal,Chwedyk Hub,Rusch Landing"
+ },
+ {
+ "name": "Bets",
+ "pos_x": 150.96875,
+ "pos_y": -65.40625,
+ "pos_z": -16.03125,
+ "stations": "Panshin Dock,Kippax Colony,Stuart Colony,Hottot Prospect"
+ },
+ {
+ "name": "Betse Aibes",
+ "pos_x": 26.0625,
+ "pos_y": 116.125,
+ "pos_z": -53.25,
+ "stations": "Williams Station,Leinster Colony,Griffith Arsenal,Moran Works"
+ },
+ {
+ "name": "Betse Eyo",
+ "pos_x": -14.5,
+ "pos_y": -107.96875,
+ "pos_z": -31.15625,
+ "stations": "Skiff Enterprise,Ejigu Enterprise,Alcock Plant"
+ },
+ {
+ "name": "Betseoltana",
+ "pos_x": 19.78125,
+ "pos_y": 117.375,
+ "pos_z": -82.625,
+ "stations": "Rushworth Colony,Henricks Port,Heceta Base,Rorschach Survey"
+ },
+ {
+ "name": "Bevan's Hope",
+ "pos_x": 68.71875,
+ "pos_y": 5,
+ "pos_z": 28.4375,
+ "stations": "Peters Depot"
+ },
+ {
+ "name": "BF Canis Venatici",
+ "pos_x": -8.25,
+ "pos_y": 62.1875,
+ "pos_z": -3.0625,
+ "stations": "Jordan Hub,Cramer Plant,Stapledon Point"
+ },
+ {
+ "name": "Bhada",
+ "pos_x": 64.03125,
+ "pos_y": -35.8125,
+ "pos_z": 8.84375,
+ "stations": "Resnick Settlement"
+ },
+ {
+ "name": "Bhadaba",
+ "pos_x": -18.96875,
+ "pos_y": -19.40625,
+ "pos_z": -49.78125,
+ "stations": "Ayerdhal City,Nakaya Works,Humphreys Enterprise,Derleth Orbital,Dedman Gateway,Polya Enterprise,Reis Prospect,Roberts Hub,Bailey Ring,Maire Gateway,Cavendish Prospect,Gantt Legacy,Godel Legacy,Kandel Ring"
+ },
+ {
+ "name": "Bhadal",
+ "pos_x": 107.5,
+ "pos_y": -188.03125,
+ "pos_z": 18.75,
+ "stations": "Bohme Outpost,Davies Survey,Laumer Landing"
+ },
+ {
+ "name": "Bhadi",
+ "pos_x": 158.375,
+ "pos_y": -116.9375,
+ "pos_z": 18.28125,
+ "stations": "Kamov Settlement,Kraft Hub"
+ },
+ {
+ "name": "Bhado",
+ "pos_x": -114.8125,
+ "pos_y": -107.75,
+ "pos_z": 24.875,
+ "stations": "Rasmussen Enterprise,Napier Station,Shawl Arsenal,Carpini Prospect"
+ },
+ {
+ "name": "Bhadra",
+ "pos_x": -76.9375,
+ "pos_y": -71.125,
+ "pos_z": -9.25,
+ "stations": "Frimout Gateway,Tereshkova Station,Whitney Orbital,Novitski Works,Whitney's Claim"
+ },
+ {
+ "name": "Bhaga",
+ "pos_x": -23.65625,
+ "pos_y": 45.84375,
+ "pos_z": -155.8125,
+ "stations": "Schiltberger Dock,Barnes Vision,Meaney Dock,Hugh Enterprise,O'Brien Penal colony"
+ },
+ {
+ "name": "Bhagga",
+ "pos_x": -116,
+ "pos_y": 15.21875,
+ "pos_z": 101.96875,
+ "stations": "Gordon's Folly,Barcelos Arena"
+ },
+ {
+ "name": "Bhaggara",
+ "pos_x": 120.53125,
+ "pos_y": -19.4375,
+ "pos_z": -32.6875,
+ "stations": "Schouten Asylum,MacDonald Vista"
+ },
+ {
+ "name": "Bhagiriyot",
+ "pos_x": 144.84375,
+ "pos_y": -210.75,
+ "pos_z": -57.875,
+ "stations": "Garrido Colony"
+ },
+ {
+ "name": "Bhagites",
+ "pos_x": 73.8125,
+ "pos_y": 93,
+ "pos_z": -4.6875,
+ "stations": "Gurragchaa Landing,Forsskal Works,Singer Landing"
+ },
+ {
+ "name": "Bhagui",
+ "pos_x": 69.59375,
+ "pos_y": 62.5625,
+ "pos_z": 124.46875,
+ "stations": "Leavitt Port,Ingstad Base,Humphreys Vista"
+ },
+ {
+ "name": "Bhaguru",
+ "pos_x": -4.84375,
+ "pos_y": 96.90625,
+ "pos_z": -60.59375,
+ "stations": "Kandel Terminal,Alpers Gateway"
+ },
+ {
+ "name": "Bhaguthians",
+ "pos_x": 110.375,
+ "pos_y": 87.5,
+ "pos_z": 9.09375,
+ "stations": "Al-Battani Base"
+ },
+ {
+ "name": "Bhaguti",
+ "pos_x": 41.6875,
+ "pos_y": 36.3125,
+ "pos_z": 18.9375,
+ "stations": "Fleming Holdings,Maxwell Hub,Mondeh Holdings"
+ },
+ {
+ "name": "Bhaguting",
+ "pos_x": 116.78125,
+ "pos_y": -189.78125,
+ "pos_z": 72.25,
+ "stations": "Schwabe Settlement"
+ },
+ {
+ "name": "Bhagutsuk",
+ "pos_x": -36.6875,
+ "pos_y": -12.84375,
+ "pos_z": -120.46875,
+ "stations": "Gardner Hub,Grzimek Point,Auld Beacon"
+ },
+ {
+ "name": "Bhaidenwi",
+ "pos_x": -94.21875,
+ "pos_y": 44.40625,
+ "pos_z": 17.5,
+ "stations": "Crowley Terminal,Murdoch Beacon,Vancouver Settlement"
+ },
+ {
+ "name": "Bhaimyr",
+ "pos_x": -18.28125,
+ "pos_y": -207.15625,
+ "pos_z": 12.5625,
+ "stations": "Kojima Port,Roemer Prospect,Wallace Settlement"
+ },
+ {
+ "name": "Bhairmat",
+ "pos_x": -128.6875,
+ "pos_y": 54.0625,
+ "pos_z": 24.15625,
+ "stations": "Boming Gateway,Magnus Hub,Viktorenko Platform,Samokutyayev Vision,Moore Mines"
+ },
+ {
+ "name": "Bhaja",
+ "pos_x": -64.125,
+ "pos_y": -84.9375,
+ "pos_z": -33.78125,
+ "stations": "Szebehely Prospect,Barratt Mines"
+ },
+ {
+ "name": "Bhajaja",
+ "pos_x": 101.625,
+ "pos_y": -78.84375,
+ "pos_z": -4.3125,
+ "stations": "Cowper's Inheritance,Apianus Refinery,Wegner Hub"
+ },
+ {
+ "name": "Bhajeri",
+ "pos_x": 37.15625,
+ "pos_y": -121.53125,
+ "pos_z": 96.0625,
+ "stations": "Lubbock Enterprise,Abel Enterprise,Giacobini Survey"
+ },
+ {
+ "name": "Bhal",
+ "pos_x": -87.03125,
+ "pos_y": -71.6875,
+ "pos_z": -134.65625,
+ "stations": "Armstrong Enterprise,Vaucanson Installation,Fulton Prospect,Thomson Orbital,Gauss Station,Rescue Ship - Armstrong Enterprise"
+ },
+ {
+ "name": "Bhalan",
+ "pos_x": 163.09375,
+ "pos_y": -136.125,
+ "pos_z": -58,
+ "stations": "Payne-Gaposchkin Survey,Wingrove Relay,McCrea Hub,Zoline Prospect"
+ },
+ {
+ "name": "Bhalanas",
+ "pos_x": 165.78125,
+ "pos_y": -164.59375,
+ "pos_z": 53.84375,
+ "stations": "Murakami Port,Napier Installation"
+ },
+ {
+ "name": "Bhalenses",
+ "pos_x": -63.625,
+ "pos_y": -44,
+ "pos_z": 48.9375,
+ "stations": "Makeev Outpost,Ledyard Bastion"
+ },
+ {
+ "name": "Bhaluwa",
+ "pos_x": -93.6875,
+ "pos_y": -26.09375,
+ "pos_z": 105.4375,
+ "stations": "Murphy Silo,Pettit Terminal,Morey Landing,Svavarsson Laboratory"
+ },
+ {
+ "name": "Bharaba",
+ "pos_x": 121.875,
+ "pos_y": -91.5625,
+ "pos_z": 75,
+ "stations": "Helffrich Station,Tasman Exchange,Ryan Hub,Pennington Port,Walter Port,Hubble Ring,Malchiodi Landing,Espenak Terminal,Evans Settlement,Allen Hub,Danforth Terminal"
+ },
+ {
+ "name": "Bharani",
+ "pos_x": -65.21875,
+ "pos_y": -78.03125,
+ "pos_z": -130.78125,
+ "stations": "Shukor Depot,Parazynski Works,Marshall Refinery"
+ },
+ {
+ "name": "Bhare",
+ "pos_x": 10.3125,
+ "pos_y": -86.96875,
+ "pos_z": 97.0625,
+ "stations": "Pacheco Orbital,Rabinowitz Vision,Adams Terminal,Tsunenaga Camp,Vercors Installation"
+ },
+ {
+ "name": "Bharezo",
+ "pos_x": 2.78125,
+ "pos_y": -24.84375,
+ "pos_z": -119.375,
+ "stations": "Northrop Holdings,Howard Enterprise,Still Orbital"
+ },
+ {
+ "name": "Bhariaborii",
+ "pos_x": 56.65625,
+ "pos_y": 60.125,
+ "pos_z": -126.09375,
+ "stations": "Bramah Terminal,Dezhurov Point,MacCurdy Terminal"
+ },
+ {
+ "name": "Bharini",
+ "pos_x": 23.78125,
+ "pos_y": 50.875,
+ "pos_z": -89.5,
+ "stations": "Neville Prospect,Petaja Estate,Clapperton Terminal,Kozlov Settlement"
+ },
+ {
+ "name": "Bharu",
+ "pos_x": 67.25,
+ "pos_y": 78.84375,
+ "pos_z": 21.1875,
+ "stations": "Xiaoguan Installation,Roberts Silo"
+ },
+ {
+ "name": "Bhatas",
+ "pos_x": 115.5,
+ "pos_y": -46.84375,
+ "pos_z": 5.15625,
+ "stations": "Brundage Gateway,Wachmann City,Cowell Station,Urata City,Gloss Hub,Niijima Ring,Reichelt City,Pickering Dock,Friedman Orbital,Furukawa Station,Rand Camp"
+ },
+ {
+ "name": "Bhati",
+ "pos_x": -96.125,
+ "pos_y": 4.125,
+ "pos_z": 155.3125,
+ "stations": "Campbell Enterprise,Tem Arena,Russell Base"
+ },
+ {
+ "name": "Bhatikan",
+ "pos_x": 68.53125,
+ "pos_y": -57.40625,
+ "pos_z": 135.84375,
+ "stations": "Palisa Stop"
+ },
+ {
+ "name": "Bhattra",
+ "pos_x": -90.125,
+ "pos_y": -54.375,
+ "pos_z": 95.125,
+ "stations": "Levinson Orbital,Stasheff Hub,Kube-McDowell Horizons,Galindo Orbital,Bruce Holdings"
+ },
+ {
+ "name": "Bhava",
+ "pos_x": 46.875,
+ "pos_y": 5.03125,
+ "pos_z": -158.5625,
+ "stations": "Gagarin Orbital,Mitchell Terminal,vo Colony,Tapinas Prospect,Lichtenberg Landing"
+ },
+ {
+ "name": "Bhavanates",
+ "pos_x": -98.46875,
+ "pos_y": -1.5,
+ "pos_z": 88.8125,
+ "stations": "Akers Platform"
+ },
+ {
+ "name": "Bhavas",
+ "pos_x": 138.1875,
+ "pos_y": -103.5625,
+ "pos_z": -41.96875,
+ "stations": "Sugano Orbital,Schuster Terminal,Samos Port,de Andrade Barracks,Laing Horizons"
+ },
+ {
+ "name": "Bhil",
+ "pos_x": 149.78125,
+ "pos_y": -36.53125,
+ "pos_z": -12.9375,
+ "stations": "Cassini Dock"
+ },
+ {
+ "name": "Bhil Mina",
+ "pos_x": 59.1875,
+ "pos_y": 1.65625,
+ "pos_z": 41.625,
+ "stations": "Halley Terminal,Mohmand Gateway,Sturckow Dock,Rennie Orbital,McArthur Port,Bykovsky Station,Hieb City,Thuot Port,Daniel Horizons,Zholobov Terminal,LeConte Landing,Raleigh Depot"
+ },
+ {
+ "name": "Bhilinool",
+ "pos_x": 121.5,
+ "pos_y": -115.34375,
+ "pos_z": 60.40625,
+ "stations": "Lee Port,Tousey Barracks,Hencke Vision"
+ },
+ {
+ "name": "Bhillke",
+ "pos_x": -0.59375,
+ "pos_y": -83.09375,
+ "pos_z": -137.21875,
+ "stations": "Brahe Depot,Curie Mines,Phillips Relay"
+ },
+ {
+ "name": "Bhilonggam",
+ "pos_x": 191.6875,
+ "pos_y": -167.9375,
+ "pos_z": 30.40625,
+ "stations": "Foglio Camp,Adams Works"
+ },
+ {
+ "name": "Bhime",
+ "pos_x": 62.96875,
+ "pos_y": 36.90625,
+ "pos_z": -149.15625,
+ "stations": "Thoreau Terminal,Messerschmid Dock,Berezovoy Ring"
+ },
+ {
+ "name": "Bhisara",
+ "pos_x": 79.8125,
+ "pos_y": -181.6875,
+ "pos_z": 119.84375,
+ "stations": "Very Station"
+ },
+ {
+ "name": "Bhisas",
+ "pos_x": -79.4375,
+ "pos_y": 100.53125,
+ "pos_z": 8.59375,
+ "stations": "Nachtigal Platform,Corte-Real Horizons,Hui Gateway"
+ },
+ {
+ "name": "Bhisma",
+ "pos_x": 51.5625,
+ "pos_y": -74.1875,
+ "pos_z": -137.125,
+ "stations": "Dupuy de Lome Dock"
+ },
+ {
+ "name": "Bhodha",
+ "pos_x": 17.96875,
+ "pos_y": -82.34375,
+ "pos_z": 80.8125,
+ "stations": "Coles Penal colony,Harawi Terminal"
+ },
+ {
+ "name": "bhodumba",
+ "pos_x": -123.0625,
+ "pos_y": -3.28125,
+ "pos_z": -114.65625,
+ "stations": "Herreshoff Legacy,Anthony de la Roche's Progress"
+ },
+ {
+ "name": "Bhote",
+ "pos_x": 44.6875,
+ "pos_y": -167.40625,
+ "pos_z": -86.96875,
+ "stations": "Wisdom Survey"
+ },
+ {
+ "name": "Bhotega",
+ "pos_x": -55.21875,
+ "pos_y": 14.5,
+ "pos_z": -25.15625,
+ "stations": "Savitskaya Ring,Voss Ring,Karl Diesel Dock"
+ },
+ {
+ "name": "Bhoteganui",
+ "pos_x": -17.3125,
+ "pos_y": -138,
+ "pos_z": 76.25,
+ "stations": "Naboth Terminal,Kowal Vision,Pons Orbital,Chernykh Base,Spielberg Base"
+ },
+ {
+ "name": "Bhotenoi",
+ "pos_x": 22.25,
+ "pos_y": -233.15625,
+ "pos_z": -21.09375,
+ "stations": "Louis de Lacaille Landing"
+ },
+ {
+ "name": "Bhotepa",
+ "pos_x": -109.15625,
+ "pos_y": 35.96875,
+ "pos_z": -59.375,
+ "stations": "Meitner Dock,Klimuk Plant"
+ },
+ {
+ "name": "Bhotepago",
+ "pos_x": 156.21875,
+ "pos_y": -16.15625,
+ "pos_z": -57.5625,
+ "stations": "Carpenter Barracks,Hermaszewski Orbital,la Cosa Installation"
+ },
+ {
+ "name": "Bhotho",
+ "pos_x": -65.375,
+ "pos_y": -19.40625,
+ "pos_z": 86.53125,
+ "stations": "Lorentz Dock,Baker Holdings,Brand Hub,Clute Prospect"
+ },
+ {
+ "name": "Bhottada",
+ "pos_x": -113.28125,
+ "pos_y": 69.96875,
+ "pos_z": -13.75,
+ "stations": "Kwolek Mines,Artin Barracks"
+ },
+ {
+ "name": "Bhottadja",
+ "pos_x": -143.96875,
+ "pos_y": -71.0625,
+ "pos_z": 39.40625,
+ "stations": "McMullen Survey,Cavendish Observatory"
+ },
+ {
+ "name": "Bhrige",
+ "pos_x": -118.46875,
+ "pos_y": 63.53125,
+ "pos_z": 105.0625,
+ "stations": "Hayden Dock"
+ },
+ {
+ "name": "Bhrima",
+ "pos_x": 55.75,
+ "pos_y": 88.625,
+ "pos_z": 70.1875,
+ "stations": "Savitskaya Hub,Vaugh City,Benz Gateway,Fullerton Ring,Jacquard Terminal"
+ },
+ {
+ "name": "Bhrites",
+ "pos_x": 120.09375,
+ "pos_y": -194.625,
+ "pos_z": 70.625,
+ "stations": "Christy's Pride,Hirn Base"
+ },
+ {
+ "name": "Bhritzameno",
+ "pos_x": -11.3125,
+ "pos_y": -1.03125,
+ "pos_z": 15.34375,
+ "stations": "Feynman Terminal,Giles Keep,Froude Camp,Dalton City"
+ },
+ {
+ "name": "Bhumato",
+ "pos_x": -115.125,
+ "pos_y": 76.875,
+ "pos_z": -109.125,
+ "stations": "Filipchenko Platform,Casper Plant"
+ },
+ {
+ "name": "Bhumatz",
+ "pos_x": -67.90625,
+ "pos_y": 40.0625,
+ "pos_z": 17.0625,
+ "stations": "Cabrera Terminal,Chaudhary Dock"
+ },
+ {
+ "name": "Bhumbi",
+ "pos_x": 78.6875,
+ "pos_y": -64.53125,
+ "pos_z": 63.46875,
+ "stations": "Westphal Hangar,Kraft Plant,Nakamura Mines"
+ },
+ {
+ "name": "Bhumbla",
+ "pos_x": -118.6875,
+ "pos_y": 76.78125,
+ "pos_z": 27.9375,
+ "stations": "Garriott Beacon"
+ },
+ {
+ "name": "Bhumian",
+ "pos_x": 108.65625,
+ "pos_y": -250.71875,
+ "pos_z": 93.28125,
+ "stations": "Hansteen Orbital,Nomura Prospect"
+ },
+ {
+ "name": "Bhumian Ku",
+ "pos_x": -42.53125,
+ "pos_y": -7.03125,
+ "pos_z": -127.03125,
+ "stations": "Ballard Ring,Levy Relay,Brooks Keep,Vancouver Hub"
+ },
+ {
+ "name": "Bhumians",
+ "pos_x": -60.78125,
+ "pos_y": 139.4375,
+ "pos_z": 35.03125,
+ "stations": "Steinmuller Dock,Kelleam Bastion"
+ },
+ {
+ "name": "Bhumij",
+ "pos_x": 68.625,
+ "pos_y": -137.4375,
+ "pos_z": -19.6875,
+ "stations": "Vyssotsky Mines,Stone Dock,Blish Arsenal"
+ },
+ {
+ "name": "Bhumijaban",
+ "pos_x": -61.5625,
+ "pos_y": -6.65625,
+ "pos_z": 157.125,
+ "stations": "Pohl's Exile,RenenBellot Stop"
+ },
+ {
+ "name": "Bhumisii",
+ "pos_x": 110.90625,
+ "pos_y": -136.875,
+ "pos_z": 32.71875,
+ "stations": "Chandra Landing,Hoffmeister Enterprise"
+ },
+ {
+ "name": "Bhumliauri",
+ "pos_x": 118.90625,
+ "pos_y": 70.78125,
+ "pos_z": 110.09375,
+ "stations": "Ferguson's Exile"
+ },
+ {
+ "name": "Bhumliaurt",
+ "pos_x": -163.78125,
+ "pos_y": -20.71875,
+ "pos_z": 40.34375,
+ "stations": "Lichtenberg Port,Tsunenaga Dock,West Survey"
+ },
+ {
+ "name": "Bhun Chi",
+ "pos_x": 147.34375,
+ "pos_y": -108.4375,
+ "pos_z": 131.375,
+ "stations": "Conti Base,Winthrop Beacon"
+ },
+ {
+ "name": "Bhunai",
+ "pos_x": -53.28125,
+ "pos_y": -73.34375,
+ "pos_z": 93.28125,
+ "stations": "Rothfuss Dock,Hume Point"
+ },
+ {
+ "name": "Bhungkan",
+ "pos_x": 158.3125,
+ "pos_y": 39.1875,
+ "pos_z": 31.125,
+ "stations": "Franke Camp,Peary Refinery,Kozeyev Vision"
+ },
+ {
+ "name": "Bhutas",
+ "pos_x": 86.4375,
+ "pos_y": 51,
+ "pos_z": 91.0625,
+ "stations": "Ciferri Holdings,Feynman Terminal,Ohm Platform,Lavrador Installation,Ryman Horizons"
+ },
+ {
+ "name": "Bhutatani",
+ "pos_x": 113.3125,
+ "pos_y": -15.0625,
+ "pos_z": 23.46875,
+ "stations": "Ings Holdings,Kennedy Settlement,Brooks Port"
+ },
+ {
+ "name": "Bhutie",
+ "pos_x": 61.03125,
+ "pos_y": -102.84375,
+ "pos_z": 79.21875,
+ "stations": "Gabrielli Station"
+ },
+ {
+ "name": "Bi Dhoraja",
+ "pos_x": 158.75,
+ "pos_y": 48.1875,
+ "pos_z": 41.84375,
+ "stations": "Bendell Port"
+ },
+ {
+ "name": "Bi Dhorora",
+ "pos_x": 167.90625,
+ "pos_y": -95.4375,
+ "pos_z": -42.0625,
+ "stations": "Rolland Installation,Shea Relay"
+ },
+ {
+ "name": "Biambar",
+ "pos_x": 112.5,
+ "pos_y": -241.90625,
+ "pos_z": 25.5625,
+ "stations": "Baldwin Vision"
+ },
+ {
+ "name": "Biamebitoto",
+ "pos_x": 53.28125,
+ "pos_y": -0.21875,
+ "pos_z": 118.1875,
+ "stations": "Goddard Hub,Euclid Terminal"
+ },
+ {
+ "name": "Biamoana",
+ "pos_x": -139.25,
+ "pos_y": -45.59375,
+ "pos_z": 39.6875,
+ "stations": "Shargin Landing,Artyukhin Port,Comer Settlement"
+ },
+ {
+ "name": "Biaris",
+ "pos_x": -68.5625,
+ "pos_y": -43.125,
+ "pos_z": 12.34375,
+ "stations": "Gamow Port,Moskowitz Silo,Noriega Mines"
+ },
+ {
+ "name": "Biarnun",
+ "pos_x": -112.4375,
+ "pos_y": 111.90625,
+ "pos_z": -7.875,
+ "stations": "MacLean Mine"
+ },
+ {
+ "name": "Biatae",
+ "pos_x": 58.5,
+ "pos_y": -78.59375,
+ "pos_z": 174.09375,
+ "stations": "Phillifent Camp"
+ },
+ {
+ "name": "Biataxo",
+ "pos_x": 23.15625,
+ "pos_y": 67.875,
+ "pos_z": -27.4375,
+ "stations": "Volta's Progress,Macquorn Rankine Retreat"
+ },
+ {
+ "name": "Biatelara",
+ "pos_x": -30.75,
+ "pos_y": -70.6875,
+ "pos_z": 120.15625,
+ "stations": "Kapteyn Enterprise"
+ },
+ {
+ "name": "Biballi",
+ "pos_x": 168.25,
+ "pos_y": -115.90625,
+ "pos_z": -56.78125,
+ "stations": "Stephan City,Titius Ring,Altuna Ring,Flynn Prospect,Tully Hub"
+ },
+ {
+ "name": "Bibambiu",
+ "pos_x": 132,
+ "pos_y": -10.25,
+ "pos_z": 41.21875,
+ "stations": "Alexandria Port"
+ },
+ {
+ "name": "Bibari",
+ "pos_x": 79.15625,
+ "pos_y": -159.03125,
+ "pos_z": 55.625,
+ "stations": "Altuna Hub,Kennedy's Inheritance,Frazetta Vision"
+ },
+ {
+ "name": "Bibaridji",
+ "pos_x": -104.875,
+ "pos_y": -122.65625,
+ "pos_z": -58.3125,
+ "stations": "Sanger Port,Akers Installation"
+ },
+ {
+ "name": "Bibato",
+ "pos_x": 167.65625,
+ "pos_y": -157.71875,
+ "pos_z": -7.65625,
+ "stations": "Chertovsky Vision,Dassault Hub,Wisniewski-Snerg Point"
+ },
+ {
+ "name": "Bibatolak",
+ "pos_x": 110.40625,
+ "pos_y": 17.71875,
+ "pos_z": -49.6875,
+ "stations": "Melroy Hub,Rashid Platform,Lovell Point"
+ },
+ {
+ "name": "Bibri",
+ "pos_x": -114.96875,
+ "pos_y": -99.625,
+ "pos_z": -60.09375,
+ "stations": "Maire Port,Guerrero Port,Weyl Orbital,Leestma Arsenal"
+ },
+ {
+ "name": "Bibrigen",
+ "pos_x": 29.125,
+ "pos_y": 97.90625,
+ "pos_z": 97.09375,
+ "stations": "Jacobi Co-operative,Eudoxus Dock,Barjavel Orbital,Griffin Hub,Leinster Stop"
+ },
+ {
+ "name": "Bibriges",
+ "pos_x": 90.125,
+ "pos_y": -0.09375,
+ "pos_z": 145.09375,
+ "stations": "Filter Plant,Semeonis Beacon,Steiner Plant"
+ },
+ {
+ "name": "Bibrocorna",
+ "pos_x": -58.3125,
+ "pos_y": 72.40625,
+ "pos_z": -94.09375,
+ "stations": "Sarrantonio Co-operative,la Cosa Enterprise,Brosnan City,Karlsefni Plant,Bixby Keep"
+ },
+ {
+ "name": "Bida",
+ "pos_x": -82.78125,
+ "pos_y": -42.59375,
+ "pos_z": -24.46875,
+ "stations": "Leopold Base,Meikle Enterprise"
+ },
+ {
+ "name": "Bidal",
+ "pos_x": 0.8125,
+ "pos_y": -134.8125,
+ "pos_z": 100.125,
+ "stations": "Al Sufi Mine,Moore Mines"
+ },
+ {
+ "name": "Bidawal",
+ "pos_x": 57.375,
+ "pos_y": -31.65625,
+ "pos_z": -85.71875,
+ "stations": "Shkaplerov Colony,Garratt Orbital"
+ },
+ {
+ "name": "Bidia",
+ "pos_x": -58.5,
+ "pos_y": -41.125,
+ "pos_z": 151.84375,
+ "stations": "Moresby City,Lerner Installation,Williamson Gateway"
+ },
+ {
+ "name": "Bidiae",
+ "pos_x": -138.28125,
+ "pos_y": -60.15625,
+ "pos_z": 8.78125,
+ "stations": "Heyerdahl Co-operative,Stross' Progress,Kent Hub,Cremona Point,Brunner Dock,Anthony de la Roche Plant"
+ },
+ {
+ "name": "Bidio",
+ "pos_x": -79.8125,
+ "pos_y": -14.625,
+ "pos_z": -86.65625,
+ "stations": "Parry's Inheritance,Noguchi Horizons,Boswell Orbital,Beliaev's Pride,Elion Horizons"
+ },
+ {
+ "name": "Bidiono",
+ "pos_x": 102.96875,
+ "pos_y": 18.8125,
+ "pos_z": 64.53125,
+ "stations": "Wellman Dock,Jemison Beacon,Mastracchio Beacon,Kubasov Settlement,Brahe Ring,Guidoni Colony"
+ },
+ {
+ "name": "Bidja",
+ "pos_x": -40.5,
+ "pos_y": -43.875,
+ "pos_z": 19.59375,
+ "stations": "Foucault Colony"
+ },
+ {
+ "name": "Bidjanapu",
+ "pos_x": 43.03125,
+ "pos_y": 56.5,
+ "pos_z": 78.8125,
+ "stations": "Elgin Settlement,Quick Holdings,Nehsi Hangar"
+ },
+ {
+ "name": "Bidjimba",
+ "pos_x": 70.5625,
+ "pos_y": -118.78125,
+ "pos_z": -66.40625,
+ "stations": "Eddington Dock,Endate Port,Reinhold Port,Felice Depot,Ledyard Prospect,Le Guin Reach"
+ },
+ {
+ "name": "Bidjogo",
+ "pos_x": -49.46875,
+ "pos_y": -137,
+ "pos_z": 51.5625,
+ "stations": "Ivanishin Station,Fincke Settlement,Delbruck Vision"
+ },
+ {
+ "name": "Biela",
+ "pos_x": -153.5,
+ "pos_y": 71.34375,
+ "pos_z": 32.375,
+ "stations": "Cabana Ring,Euclid Gateway,Arrhenius Dock,Vries Hub"
+ },
+ {
+ "name": "Bielegua",
+ "pos_x": -63.21875,
+ "pos_y": 42.625,
+ "pos_z": -76.53125,
+ "stations": "Gooch Vision,Ramanujan Port"
+ },
+ {
+ "name": "Bielenses",
+ "pos_x": -145.625,
+ "pos_y": -13.46875,
+ "pos_z": 8.125,
+ "stations": "Casper Terminal,Brand Orbital,Crown Terminal,Aldrin Station,Ericsson Dock,Garcia Exchange"
+ },
+ {
+ "name": "Bielo Kakma",
+ "pos_x": -89.90625,
+ "pos_y": 75.28125,
+ "pos_z": 121.4375,
+ "stations": "Kirk Legacy"
+ },
+ {
+ "name": "Bielonti",
+ "pos_x": 25.6875,
+ "pos_y": 80.25,
+ "pos_z": 8.4375,
+ "stations": "Ahmed Dock,Gotlieb Penal colony"
+ },
+ {
+ "name": "Bieltani",
+ "pos_x": 58,
+ "pos_y": 8.40625,
+ "pos_z": 95.3125,
+ "stations": "Singer Bastion,Mitchell Enterprise,Marshburn Enterprise"
+ },
+ {
+ "name": "Bifrost",
+ "pos_x": 58.6875,
+ "pos_y": 54.28125,
+ "pos_z": -62.46875,
+ "stations": "Godwin Hangar,Tokubei Silo,Wescott Settlement"
+ },
+ {
+ "name": "Biga",
+ "pos_x": 22.21875,
+ "pos_y": -12.125,
+ "pos_z": 152.90625,
+ "stations": "Freycinet Freeport,Parry Retreat,Watt-Evans Reach"
+ },
+ {
+ "name": "Biham",
+ "pos_x": -66.59375,
+ "pos_y": -58.09375,
+ "pos_z": 26.625,
+ "stations": "Currie Camp,Waldeck Refinery"
+ },
+ {
+ "name": "Bijedeta",
+ "pos_x": 58.46875,
+ "pos_y": -7.28125,
+ "pos_z": 96.03125,
+ "stations": "Carpenter Hub,Arnold Refinery,Bentham Horizons"
+ },
+ {
+ "name": "Bijemayah",
+ "pos_x": 140.90625,
+ "pos_y": -208.75,
+ "pos_z": 25.3125,
+ "stations": "Tusi Enterprise,Muramatsu Enterprise,Nakano Dock"
+ },
+ {
+ "name": "Bikeggirina",
+ "pos_x": 33.59375,
+ "pos_y": -183.875,
+ "pos_z": 96.96875,
+ "stations": "Condit Terminal,Becvar Port,Arp's Folly,Shoemaker Enterprise"
+ },
+ {
+ "name": "Biko",
+ "pos_x": -99.96875,
+ "pos_y": -108.125,
+ "pos_z": 25.8125,
+ "stations": "Forstchen Horizons,Stjepan Seljan Settlement"
+ },
+ {
+ "name": "Bikoja",
+ "pos_x": 55.75,
+ "pos_y": 140.5,
+ "pos_z": 0.90625,
+ "stations": "Crouch Station"
+ },
+ {
+ "name": "Bikokujungu",
+ "pos_x": 122.21875,
+ "pos_y": -68.53125,
+ "pos_z": 11.125,
+ "stations": "Abnett Installation,Lagerkvist Holdings"
+ },
+ {
+ "name": "Bikoro",
+ "pos_x": 98.5625,
+ "pos_y": 40.21875,
+ "pos_z": -120,
+ "stations": "Barr Bastion,Pournelle Dock"
+ },
+ {
+ "name": "Bildans",
+ "pos_x": 16.5625,
+ "pos_y": -137.4375,
+ "pos_z": 123.9375,
+ "stations": "Endate Beacon"
+ },
+ {
+ "name": "Bildeptu",
+ "pos_x": 62.125,
+ "pos_y": -162.53125,
+ "pos_z": 72.6875,
+ "stations": "Coye Prospect"
+ },
+ {
+ "name": "Bileku",
+ "pos_x": 160.125,
+ "pos_y": -51.90625,
+ "pos_z": -40.625,
+ "stations": "Effinger Vision,Helffrich Vision,Dolgov Terminal"
+ },
+ {
+ "name": "Bilenufang",
+ "pos_x": -138.21875,
+ "pos_y": 76.46875,
+ "pos_z": 19.25,
+ "stations": "Chaviano Landing,Lopez de Haro Point"
+ },
+ {
+ "name": "Bilfrost",
+ "pos_x": -10.28125,
+ "pos_y": 100.90625,
+ "pos_z": -0.65625,
+ "stations": "Williams Port,Auer Orbital,Scithers Ring,Bear Hub,Gromov Port,Godwin Hub,Busch Landing,Kornbluth Gateway,Kennan Station,Alexeyev Orbital,Chandler Enterprise,Xuanzang Installation,Burkin Works,Bass Installation"
+ },
+ {
+ "name": "Bilingara",
+ "pos_x": -8.96875,
+ "pos_y": -78.375,
+ "pos_z": 112.46875,
+ "stations": "Shawl Point"
+ },
+ {
+ "name": "Biliri",
+ "pos_x": 85.65625,
+ "pos_y": -58.625,
+ "pos_z": -61.125,
+ "stations": "Fan City,Stjepan Seljan Barracks"
+ },
+ {
+ "name": "Bilobor",
+ "pos_x": 49.375,
+ "pos_y": -129.28125,
+ "pos_z": 77.875,
+ "stations": "Kurtz Colony"
+ },
+ {
+ "name": "Bilonesses",
+ "pos_x": 63.4375,
+ "pos_y": 119.25,
+ "pos_z": -25.375,
+ "stations": "Tanaka Hub,Vasquez de Coronado Terminal,Arthur Orbital,Bujold Enterprise,Linge Enterprise,Ford Hub,Noli Dock,Godel Terminal,White Port,Wright Relay,Glenn's Claim,Townshend's Inheritance,Miletus Terminal,Timofeyevich Prospect"
+ },
+ {
+ "name": "Bilongo",
+ "pos_x": 104.65625,
+ "pos_y": 102.6875,
+ "pos_z": 27.1875,
+ "stations": "Porges Landing,Finney Dock,Kingsmill Colony"
+ },
+ {
+ "name": "Bilskirnir",
+ "pos_x": 77.15625,
+ "pos_y": 15.03125,
+ "pos_z": -64.3125,
+ "stations": "Fischer Ring,Ito Gateway,von Helmont Station,Lem Depot,Morey Enterprise"
+ },
+ {
+ "name": "Bina",
+ "pos_x": 166.25,
+ "pos_y": -87.3125,
+ "pos_z": 24.34375,
+ "stations": "Hubble Terminal,Jones Settlement,Nagata Terminal"
+ },
+ {
+ "name": "Binar",
+ "pos_x": 124.15625,
+ "pos_y": -14.65625,
+ "pos_z": 22.5,
+ "stations": "Ferguson Dock,Hornblower Gateway,Shaw Ring,Isherwood City,Hennen Station,Petaja Plant,Arnarson Prospect,Collins' Pride"
+ },
+ {
+ "name": "Binbara",
+ "pos_x": 141.03125,
+ "pos_y": 34.375,
+ "pos_z": 32.46875,
+ "stations": "Lamarck Gateway,Curie Gateway"
+ },
+ {
+ "name": "Binbine",
+ "pos_x": -53.78125,
+ "pos_y": 50.53125,
+ "pos_z": 55.625,
+ "stations": "Springer Survey,Gubarev Holdings,Onizuka Landing"
+ },
+ {
+ "name": "Binde",
+ "pos_x": -0.46875,
+ "pos_y": -2.34375,
+ "pos_z": 119.59375,
+ "stations": "Whitney Hub,Wang Terminal,Afanasyev Ring,Hodgkin Dock,Hudson Vista,Pudwill Gorie Legacy,Kingsmill's Inheritance"
+ },
+ {
+ "name": "Bindi",
+ "pos_x": 99.78125,
+ "pos_y": -27.96875,
+ "pos_z": 51,
+ "stations": "Bainbridge Station,Turner Horizons,Macgregor's Folly"
+ },
+ {
+ "name": "Bindjina",
+ "pos_x": 152.96875,
+ "pos_y": -139,
+ "pos_z": 93.71875,
+ "stations": "Vyssotsky Station,Merril Installation,Shaver Gateway"
+ },
+ {
+ "name": "Bingait",
+ "pos_x": -34.21875,
+ "pos_y": -7.53125,
+ "pos_z": -153.65625,
+ "stations": "Abernathy Refinery,Remec Beacon"
+ },
+ {
+ "name": "Bingo",
+ "pos_x": 16.90625,
+ "pos_y": -182.8125,
+ "pos_z": -0.78125,
+ "stations": "Hamuy Dock"
+ },
+ {
+ "name": "Bingongina",
+ "pos_x": 19.90625,
+ "pos_y": 5.4375,
+ "pos_z": -134.71875,
+ "stations": "Ray Beacon,Barbaro Stop,Dampier Hub"
+ },
+ {
+ "name": "Bingui",
+ "pos_x": 59.125,
+ "pos_y": 38.5,
+ "pos_z": -4.625,
+ "stations": "Lambert Camp,Schiltberger Hub,Tall Installation"
+ },
+ {
+ "name": "Binjakarex",
+ "pos_x": 41.40625,
+ "pos_y": 55.21875,
+ "pos_z": -114.125,
+ "stations": "Varley Mines"
+ },
+ {
+ "name": "Binjamingi",
+ "pos_x": 80.59375,
+ "pos_y": 16.15625,
+ "pos_z": -51.46875,
+ "stations": "Balandin Arena,Stafford City,Barnes Bastion,Auld Enterprise"
+ },
+ {
+ "name": "Binjanha",
+ "pos_x": -137.84375,
+ "pos_y": -10.5625,
+ "pos_z": -66.78125,
+ "stations": "Carter Port"
+ },
+ {
+ "name": "Binjhwar",
+ "pos_x": 87.875,
+ "pos_y": -172.53125,
+ "pos_z": -76.78125,
+ "stations": "Weaver Mine,Dayuan Reformatory"
+ },
+ {
+ "name": "Binjhwarku",
+ "pos_x": -104.75,
+ "pos_y": -71.53125,
+ "pos_z": 9.21875,
+ "stations": "Elion Beacon,Arkwright Mines"
+ },
+ {
+ "name": "Binjia",
+ "pos_x": 67.03125,
+ "pos_y": -49.8125,
+ "pos_z": -65.25,
+ "stations": "Curbeam Colony,Carr Orbital,Menzies Point,Planck Station"
+ },
+ {
+ "name": "Binju",
+ "pos_x": -147.5625,
+ "pos_y": -73.46875,
+ "pos_z": 33.21875,
+ "stations": "Pribylov Terminal,Malchiodi Beacon,Markov Terminal"
+ },
+ {
+ "name": "Binjuri Ku",
+ "pos_x": 97.40625,
+ "pos_y": 48.1875,
+ "pos_z": 26.90625,
+ "stations": "Arthur Mines,Chiang Relay"
+ },
+ {
+ "name": "Bipedi",
+ "pos_x": 134.6875,
+ "pos_y": -21.71875,
+ "pos_z": -11.03125,
+ "stations": "Belyanin Penal colony,Pryor Arena,Bernoulli Settlement"
+ },
+ {
+ "name": "Bipeduwa",
+ "pos_x": 57.34375,
+ "pos_y": 27.28125,
+ "pos_z": -54,
+ "stations": "Murray Vision,Torricelli Settlement,Drebbel Platform"
+ },
+ {
+ "name": "Bipek",
+ "pos_x": 20.5,
+ "pos_y": -203.9375,
+ "pos_z": 60.875,
+ "stations": "Flammarion Horizons,Baille Station,Mayer Terminal,Thollon Station,Alexander Station,Gustav Sporer Hub,Auzout Enterprise,Abasheli Bastion"
+ },
+ {
+ "name": "Bipera",
+ "pos_x": -60.875,
+ "pos_y": -105.5,
+ "pos_z": 76.03125,
+ "stations": "Tomita Port,Jung Hub"
+ },
+ {
+ "name": "Bipewyan",
+ "pos_x": -148.53125,
+ "pos_y": 1.375,
+ "pos_z": 60.40625,
+ "stations": "Chandler Sanctuary,Stairs Terminal"
+ },
+ {
+ "name": "Birangana",
+ "pos_x": 40.4375,
+ "pos_y": 64.0625,
+ "pos_z": 110.03125,
+ "stations": "Delbruck City,Wegener Hangar"
+ },
+ {
+ "name": "Birhepri",
+ "pos_x": 32.78125,
+ "pos_y": 26.0625,
+ "pos_z": 139.1875,
+ "stations": "Hovgaard Orbital"
+ },
+ {
+ "name": "Birhobo",
+ "pos_x": 75.8125,
+ "pos_y": -44.90625,
+ "pos_z": -111.5625,
+ "stations": "Hubble Colony,Binder Installation,Furukawa Orbital"
+ },
+ {
+ "name": "Birhodiweu",
+ "pos_x": -42.125,
+ "pos_y": -183.84375,
+ "pos_z": 24.90625,
+ "stations": "Hogg Port,Goldberg Station,Roed Odegaard Gateway,Mawson Settlement,Vinge Vision"
+ },
+ {
+ "name": "Birhondaker",
+ "pos_x": 164.375,
+ "pos_y": -79.1875,
+ "pos_z": -16.09375,
+ "stations": "Whitford's Inheritance,Gerlache Base"
+ },
+ {
+ "name": "Biria",
+ "pos_x": 102.125,
+ "pos_y": 56.03125,
+ "pos_z": 58.25,
+ "stations": "Chamitoff Works,Lamarck Platform"
+ },
+ {
+ "name": "Birite",
+ "pos_x": 105.625,
+ "pos_y": -86,
+ "pos_z": -81.28125,
+ "stations": "Cesar Janssen Vision,Bouvard Orbital"
+ },
+ {
+ "name": "Birjorobog",
+ "pos_x": -22.09375,
+ "pos_y": -136.5,
+ "pos_z": 61.34375,
+ "stations": "Gurney Orbital,Huss Station,Wilhelm von Struve Port,Milnor Forum"
+ },
+ {
+ "name": "Birjoromi",
+ "pos_x": -89.375,
+ "pos_y": -52.09375,
+ "pos_z": 70.71875,
+ "stations": "Somerset Platform"
+ },
+ {
+ "name": "Birjorth",
+ "pos_x": 79.75,
+ "pos_y": -13.96875,
+ "pos_z": 19.71875,
+ "stations": "Navigator Dock,Wandrei Orbital,Bloch Gateway,McMullen Enterprise,VanderMeer Dock,Schmitt Beacon,Pogue Settlement"
+ },
+ {
+ "name": "Birpinans",
+ "pos_x": 17.34375,
+ "pos_y": 24.6875,
+ "pos_z": 162.53125,
+ "stations": "Tudela's Progress,Shinn Reach,Lerman Sanctuary"
+ },
+ {
+ "name": "Birpinovit",
+ "pos_x": 129.125,
+ "pos_y": 1.625,
+ "pos_z": -67.03125,
+ "stations": "Galilei Point,Lockhart Lab"
+ },
+ {
+ "name": "Birrayak",
+ "pos_x": -130.21875,
+ "pos_y": -21.71875,
+ "pos_z": -24.8125,
+ "stations": "Curie Horizons,Boming Arsenal"
+ },
+ {
+ "name": "Birreti",
+ "pos_x": -86.5625,
+ "pos_y": -117.59375,
+ "pos_z": 29.09375,
+ "stations": "Platt City,Poisson Vision"
+ },
+ {
+ "name": "Bishama",
+ "pos_x": -2.96875,
+ "pos_y": -24.65625,
+ "pos_z": 116.75,
+ "stations": "Kroehl Terminal,White Base,Obruchev Barracks"
+ },
+ {
+ "name": "Bishamuni",
+ "pos_x": -77.53125,
+ "pos_y": -119.25,
+ "pos_z": 32.6875,
+ "stations": "White Landing,Bear Landing,Soukup Platform,Matthews Holdings"
+ },
+ {
+ "name": "Bishan",
+ "pos_x": -59.03125,
+ "pos_y": 35.34375,
+ "pos_z": -141.09375,
+ "stations": "Navigator's Inheritance,Marshburn Dock,Lister Mines,Moran Lab"
+ },
+ {
+ "name": "Bishankun",
+ "pos_x": 4.34375,
+ "pos_y": 161.5625,
+ "pos_z": 38.1875,
+ "stations": "Serling City"
+ },
+ {
+ "name": "Bishimba",
+ "pos_x": 99.28125,
+ "pos_y": 98.25,
+ "pos_z": 39.6875,
+ "stations": "Papin Horizons"
+ },
+ {
+ "name": "Bitjala",
+ "pos_x": -58.5,
+ "pos_y": -11.6875,
+ "pos_z": 96.0625,
+ "stations": "Wedge Terminal,Kopra Prospect,Hartlib Relay,Nixon Enterprise,Walker Dock,Hippalus Beacon"
+ },
+ {
+ "name": "Bjiri",
+ "pos_x": -16.09375,
+ "pos_y": 81.90625,
+ "pos_z": 139.21875,
+ "stations": "Boulton Colony"
+ },
+ {
+ "name": "Bjirup",
+ "pos_x": 55.71875,
+ "pos_y": 138.53125,
+ "pos_z": -42.96875,
+ "stations": "Kuhn Terminal,Patrick Colony"
+ },
+ {
+ "name": "Bjorora",
+ "pos_x": 105.3125,
+ "pos_y": -220.53125,
+ "pos_z": -11.03125,
+ "stations": "Consolmagno Orbital,Hoyle Colony,Charlois Oasis,Dawes Terminal"
+ },
+ {
+ "name": "Bjorovices",
+ "pos_x": -79.84375,
+ "pos_y": 38.6875,
+ "pos_z": 77.40625,
+ "stations": "Meitner Colony,Henize Port"
+ },
+ {
+ "name": "Bjorti",
+ "pos_x": 89.09375,
+ "pos_y": -175,
+ "pos_z": -62.84375,
+ "stations": "Sternbach Vision,Mainzer Enterprise,Rand Landing"
+ },
+ {
+ "name": "Bjortii",
+ "pos_x": 57.46875,
+ "pos_y": -114.25,
+ "pos_z": -101.03125,
+ "stations": "Liouville Plant,Vavrova Station,Finney Relay,McIntosh Station,Patry Orbital,Le Guin's Folly"
+ },
+ {
+ "name": "Blandagur",
+ "pos_x": 138.46875,
+ "pos_y": -211.21875,
+ "pos_z": 73.4375,
+ "stations": "Grunsfeld Reformatory,Powers Mine"
+ },
+ {
+ "name": "Blang Tzu",
+ "pos_x": -16.46875,
+ "pos_y": -167.75,
+ "pos_z": -0.03125,
+ "stations": "Consolmagno Mines,Laird's Progress,Yakovlev Platform"
+ },
+ {
+ "name": "Blango",
+ "pos_x": -66.96875,
+ "pos_y": 111.875,
+ "pos_z": -13.46875,
+ "stations": "Jacobi Settlement,Dirichlet Arsenal,Nielsen's Progress"
+ },
+ {
+ "name": "Blanquichu",
+ "pos_x": -3.15625,
+ "pos_y": -80.65625,
+ "pos_z": -79.6875,
+ "stations": "Cartwright Settlement"
+ },
+ {
+ "name": "Blata",
+ "pos_x": -152.90625,
+ "pos_y": 21.71875,
+ "pos_z": -12.6875,
+ "stations": "Hughes Penal colony,Nowak Landing,Gurragchaa Holdings,Hutton Horizons"
+ },
+ {
+ "name": "Blati",
+ "pos_x": -10.34375,
+ "pos_y": 40.1875,
+ "pos_z": -70.03125,
+ "stations": "Delbruck Installation"
+ },
+ {
+ "name": "Blatrimpe",
+ "pos_x": 29.125,
+ "pos_y": 11.3125,
+ "pos_z": -15.875,
+ "stations": "Boltzmann Hub,Pauling Orbital,Cartwright Market,Koch Beacon,Dayuan Prospect,Lee Base"
+ },
+ {
+ "name": "Blatrughna",
+ "pos_x": -129.125,
+ "pos_y": 36.125,
+ "pos_z": 15.34375,
+ "stations": "Harris Gateway,Harding Dock"
+ },
+ {
+ "name": "Blatucadrus",
+ "pos_x": 161.5,
+ "pos_y": -31.90625,
+ "pos_z": 31.96875,
+ "stations": "Wirtanen Ring,Wylie Depot"
+ },
+ {
+ "name": "Blatuntino",
+ "pos_x": 89.9375,
+ "pos_y": -126.1875,
+ "pos_z": -83.3125,
+ "stations": "Apianus Terminal,Kanai Port,Giger Port,Common Enterprise,Salgari Base"
+ },
+ {
+ "name": "Blatz",
+ "pos_x": 60.59375,
+ "pos_y": -1.5,
+ "pos_z": -47.59375,
+ "stations": "Gorbatko Enterprise,Galiano Orbital,Bentham Survey"
+ },
+ {
+ "name": "Blende",
+ "pos_x": 171.71875,
+ "pos_y": 46.0625,
+ "pos_z": 2.90625,
+ "stations": "Stackpole Survey,Robson Enterprise,Jones Horizons,Krylov Works,Katzenstein's Inheritance"
+ },
+ {
+ "name": "Blenu",
+ "pos_x": 65.9375,
+ "pos_y": 44.625,
+ "pos_z": 8.84375,
+ "stations": "Thagard Enterprise,Potrykus Orbital,Shepard Enterprise,Foda Camp,Dukaj Survey"
+ },
+ {
+ "name": "Blest",
+ "pos_x": 6.0625,
+ "pos_y": -17.75,
+ "pos_z": -33.625,
+ "stations": "Pirsan Survey,Baird Dock,Bethe Prospect,Aubakirov's Progress"
+ },
+ {
+ "name": "Bletae",
+ "pos_x": 12.5625,
+ "pos_y": -94.90625,
+ "pos_z": -159.0625,
+ "stations": "Coblentz Beacon,Vonnegut Beacon,Ellison Holdings"
+ },
+ {
+ "name": "Bletani",
+ "pos_x": -94.40625,
+ "pos_y": -95.15625,
+ "pos_z": -27.4375,
+ "stations": "Smith City,Crichton Colony,Nordenskiold Ring"
+ },
+ {
+ "name": "Bleti",
+ "pos_x": 27.40625,
+ "pos_y": -198.96875,
+ "pos_z": 75.53125,
+ "stations": "Carpenter's Progress"
+ },
+ {
+ "name": "Bletii",
+ "pos_x": 99.34375,
+ "pos_y": 6.125,
+ "pos_z": -166.71875,
+ "stations": "Besonders Port,Cabot Port,Yolen Orbital"
+ },
+ {
+ "name": "Bletones",
+ "pos_x": 59.96875,
+ "pos_y": -51.4375,
+ "pos_z": 160.40625,
+ "stations": "Wilson Colony,Hale Point"
+ },
+ {
+ "name": "Blido Piru",
+ "pos_x": 98.84375,
+ "pos_y": -93.6875,
+ "pos_z": -44.5,
+ "stations": "Miller Dock,Kanai Enterprise"
+ },
+ {
+ "name": "Blod",
+ "pos_x": 0.25,
+ "pos_y": -143.96875,
+ "pos_z": 90.4375,
+ "stations": "Calatrava Landing,Riccioli Vision,Flagg Relay"
+ },
+ {
+ "name": "Bloder",
+ "pos_x": 26.4375,
+ "pos_y": -75.21875,
+ "pos_z": 63.59375,
+ "stations": "Szebehely Station"
+ },
+ {
+ "name": "Blodes",
+ "pos_x": -26.71875,
+ "pos_y": -48.03125,
+ "pos_z": 19.25,
+ "stations": "Heng Installation,Linenger Station,Ejeta City,Parise Settlement,Stafford Keep"
+ },
+ {
+ "name": "Blodyaks",
+ "pos_x": 104.53125,
+ "pos_y": -158.21875,
+ "pos_z": 12.28125,
+ "stations": "Leonard Port,Pribylov Depot"
+ },
+ {
+ "name": "Blodyaksas",
+ "pos_x": -20.9375,
+ "pos_y": 15.15625,
+ "pos_z": 96.90625,
+ "stations": "Gohar Station,Akers Depot,Nelson Settlement"
+ },
+ {
+ "name": "Blu Thua AI-A c14-10",
+ "pos_x": -54.5,
+ "pos_y": 149.53125,
+ "pos_z": 2099.21875,
+ "stations": "Hillary Depot"
+ },
+ {
+ "name": "BO Microscopii",
+ "pos_x": -12.96875,
+ "pos_y": -90.875,
+ "pos_z": 112.03125,
+ "stations": "Pacheco Dock,Laurent Vision,Scotti Station,Godwin Installation,Fujikawa Point"
+ },
+ {
+ "name": "Boann",
+ "pos_x": 22.4375,
+ "pos_y": 48.25,
+ "pos_z": 55.9375,
+ "stations": "Edwards City"
+ },
+ {
+ "name": "Bobadzihozo",
+ "pos_x": 70.0625,
+ "pos_y": -147.5,
+ "pos_z": 5.28125,
+ "stations": "Barr Refinery,Andrews Barracks,Bolkow Colony"
+ },
+ {
+ "name": "Bobalduro",
+ "pos_x": 103.40625,
+ "pos_y": 30.125,
+ "pos_z": -117.53125,
+ "stations": "Boswell Colony,Shaw Dock"
+ },
+ {
+ "name": "Bobo",
+ "pos_x": 13.90625,
+ "pos_y": -55.28125,
+ "pos_z": 103.6875,
+ "stations": "Coleman Outpost,Clifton Reformatory"
+ },
+ {
+ "name": "Bobo Ukwus",
+ "pos_x": -20.34375,
+ "pos_y": 20.40625,
+ "pos_z": -137.53125,
+ "stations": "Olivas Hangar"
+ },
+ {
+ "name": "Bobole",
+ "pos_x": -140.4375,
+ "pos_y": -60.0625,
+ "pos_z": 18.6875,
+ "stations": "Stephenson Base"
+ },
+ {
+ "name": "Bochica",
+ "pos_x": 51.75,
+ "pos_y": -104.21875,
+ "pos_z": 91.21875,
+ "stations": "Napier Penal colony,Ziegel Orbital,Marley Vista"
+ },
+ {
+ "name": "Bodb Dearg",
+ "pos_x": 61.3125,
+ "pos_y": -99,
+ "pos_z": 61.5625,
+ "stations": "Qushji Landing,Farghani Prospect,Kanai's Folly"
+ },
+ {
+ "name": "Bodb Den",
+ "pos_x": -90.59375,
+ "pos_y": -17.09375,
+ "pos_z": 79.96875,
+ "stations": "Carr Settlement,Eisele Station"
+ },
+ {
+ "name": "Bodb Djede",
+ "pos_x": -36.46875,
+ "pos_y": 90.3125,
+ "pos_z": -68.625,
+ "stations": "Grant Enterprise,Dedman Station,Chebyshev Dock,Levi-Civita's Folly,Garfinkle Horizons"
+ },
+ {
+ "name": "Bodedi",
+ "pos_x": -14.125,
+ "pos_y": 26.40625,
+ "pos_z": -14.53125,
+ "stations": "Chwedyk Station"
+ },
+ {
+ "name": "Bodharail",
+ "pos_x": 93.28125,
+ "pos_y": -69.78125,
+ "pos_z": 27.53125,
+ "stations": "Zel'dovich Port,Albumasar Ring,MacCready Vision"
+ },
+ {
+ "name": "Bodhengue",
+ "pos_x": 94.9375,
+ "pos_y": 7.625,
+ "pos_z": -28.90625,
+ "stations": "Chretien Port,Tokarev Orbital,Dedman Landing,McIntyre Vista,Stephenson Beacon,Kondratyev Terminal,Banks Relay,Hill Landing"
+ },
+ {
+ "name": "Bodhi",
+ "pos_x": 20.15625,
+ "pos_y": -94.78125,
+ "pos_z": 137.65625,
+ "stations": "Greene Terminal"
+ },
+ {
+ "name": "Bodhinga",
+ "pos_x": 92.75,
+ "pos_y": -134.03125,
+ "pos_z": 193.125,
+ "stations": "Mather Hub,Lee Station,Ptack Orbital,Hay Forum,Wegner Arsenal"
+ },
+ {
+ "name": "Bodia",
+ "pos_x": 124.53125,
+ "pos_y": -85.125,
+ "pos_z": 132.25,
+ "stations": "Hickam Orbital,Thierree Orbital,Morrow Gateway,Deslandres Vista"
+ },
+ {
+ "name": "Bodiae",
+ "pos_x": 52.375,
+ "pos_y": -15.40625,
+ "pos_z": -142.78125,
+ "stations": "Bering Platform,Steinmuller Installation,McMullen Park,Samos Port"
+ },
+ {
+ "name": "Bodiono",
+ "pos_x": 113.84375,
+ "pos_y": -66.625,
+ "pos_z": -51.21875,
+ "stations": "Herbert Point,Olsen Settlement,Gutierrez Settlement,Ramanujan Station,Giles Vision"
+ },
+ {
+ "name": "Bodiontici",
+ "pos_x": -53.40625,
+ "pos_y": 122.90625,
+ "pos_z": 5.125,
+ "stations": "Dalmas Base,Moffitt Mine,Cummings' Inheritance"
+ },
+ {
+ "name": "Bodo",
+ "pos_x": 44.34375,
+ "pos_y": -71.46875,
+ "pos_z": 119.40625,
+ "stations": "Greene Plant,Truman Plant,Perga Oasis"
+ },
+ {
+ "name": "Boewnst KS-S c20-959",
+ "pos_x": -6195.46875,
+ "pos_y": -140.28125,
+ "pos_z": 16462.0625,
+ "stations": "Polo Harbour"
+ },
+ {
+ "name": "Boga",
+ "pos_x": -84.84375,
+ "pos_y": -3.71875,
+ "pos_z": 98.3125,
+ "stations": "Thorne Mine,Richards' Claim"
+ },
+ {
+ "name": "Bogami",
+ "pos_x": 62.15625,
+ "pos_y": 95.90625,
+ "pos_z": -87.03125,
+ "stations": "Bushkov City,Sarmiento de Gamboa Terminal,Betancourt Landing"
+ },
+ {
+ "name": "Bogati",
+ "pos_x": 47.3125,
+ "pos_y": -71.25,
+ "pos_z": 128.96875,
+ "stations": "Kurtz Hub,Phillips Landing,Froud Enterprise,Ehrenfried Kegel Landing"
+ },
+ {
+ "name": "Bogatiku",
+ "pos_x": -126.625,
+ "pos_y": 45.71875,
+ "pos_z": -12.5625,
+ "stations": "Rotsler Enterprise,Aguirre Terminal"
+ },
+ {
+ "name": "Bogatyri",
+ "pos_x": -2.65625,
+ "pos_y": 74.625,
+ "pos_z": 135.71875,
+ "stations": "Poincare Hub,Martin Outpost,Crown Observatory"
+ },
+ {
+ "name": "Bohmshohm",
+ "pos_x": -10.6875,
+ "pos_y": 20.875,
+ "pos_z": -55.8125,
+ "stations": "Huberath Settlement,Putzi OPK's Heaven,Gibson Hub,Block City"
+ },
+ {
+ "name": "Bok",
+ "pos_x": 84.40625,
+ "pos_y": -44.78125,
+ "pos_z": -21.125,
+ "stations": "Landsteiner Orbital,Bridger Landing,Schwann Port,Baxter Silo"
+ },
+ {
+ "name": "Bokip",
+ "pos_x": 80.28125,
+ "pos_y": 82.1875,
+ "pos_z": -34.1875,
+ "stations": "Moore Station,Bertin Vision,Barjavel Vision,Rochon Point"
+ },
+ {
+ "name": "Bokitoke",
+ "pos_x": 34.9375,
+ "pos_y": 26.125,
+ "pos_z": 166.59375,
+ "stations": "Wylie Landing,Key Platform,Clark Plant"
+ },
+ {
+ "name": "Bokomu",
+ "pos_x": 19.65625,
+ "pos_y": -169.96875,
+ "pos_z": 163.53125,
+ "stations": "Cuffey Port,Guerrero Base,Vlaicu Prospect"
+ },
+ {
+ "name": "Bokowatiko",
+ "pos_x": -73.03125,
+ "pos_y": 108,
+ "pos_z": -29.875,
+ "stations": "Johnson Orbital,Stott Point,Resnik Terminal,Henize Platform,Kettle Vista"
+ },
+ {
+ "name": "Bokwala",
+ "pos_x": 115.6875,
+ "pos_y": 44.71875,
+ "pos_z": -120.40625,
+ "stations": "Erdos Colony,Hiraga Orbital,Mach Landing"
+ },
+ {
+ "name": "Bolg",
+ "pos_x": -7.90625,
+ "pos_y": 34.71875,
+ "pos_z": 2.125,
+ "stations": "Mastracchio Park,Moxon's Mojo"
+ },
+ {
+ "name": "Boloca",
+ "pos_x": 62.09375,
+ "pos_y": -165.78125,
+ "pos_z": -18.03125,
+ "stations": "Dishoeck Terminal,Bassford Escape"
+ },
+ {
+ "name": "Bolocara",
+ "pos_x": 33.25,
+ "pos_y": -179,
+ "pos_z": -17.125,
+ "stations": "Herndon's Exile,Kuchemann Horizons,Littrow Colony"
+ },
+ {
+ "name": "Bolocas",
+ "pos_x": 47.875,
+ "pos_y": -195.4375,
+ "pos_z": -58.5625,
+ "stations": "Kapteyn Platform,Tikhonravov Mine"
+ },
+ {
+ "name": "Boloch",
+ "pos_x": 5.875,
+ "pos_y": -105,
+ "pos_z": 45.5,
+ "stations": "Asclepi Terminal,Endate Sanctuary,Peebles Platform"
+ },
+ {
+ "name": "Boloine",
+ "pos_x": 140.625,
+ "pos_y": -113.21875,
+ "pos_z": 95.28125,
+ "stations": "Dilworth Orbital,Hovgaard Mines,McManus Colony"
+ },
+ {
+ "name": "Bolon Huara",
+ "pos_x": -125.71875,
+ "pos_y": -17.0625,
+ "pos_z": 50.78125,
+ "stations": "McArthur Platform"
+ },
+ {
+ "name": "Bolon Tzu",
+ "pos_x": 144.46875,
+ "pos_y": -16.5,
+ "pos_z": -12.8125,
+ "stations": "Barton Enterprise,Brunel Enterprise,Dashiell Bastion"
+ },
+ {
+ "name": "Bolonaz",
+ "pos_x": -81.3125,
+ "pos_y": -116.34375,
+ "pos_z": -80.0625,
+ "stations": "Humboldt Dock"
+ },
+ {
+ "name": "Bolones",
+ "pos_x": 10.4375,
+ "pos_y": -41.375,
+ "pos_z": 75.5,
+ "stations": "Culbertson Settlement"
+ },
+ {
+ "name": "Bolonii",
+ "pos_x": 68.15625,
+ "pos_y": -138.65625,
+ "pos_z": -43.6875,
+ "stations": "Auzout Station,Frost Penal colony,Albitzky Gateway"
+ },
+ {
+ "name": "Boloo",
+ "pos_x": 11.65625,
+ "pos_y": -25.75,
+ "pos_z": 73.15625,
+ "stations": "Hale Station,Glidden Plant,Pinto Refinery,Stefanyshyn-Piper Dock"
+ },
+ {
+ "name": "Bolos",
+ "pos_x": -125.09375,
+ "pos_y": -3.8125,
+ "pos_z": 64.6875,
+ "stations": "Vizcaino Horizons,McBride Point,Rangarajan Horizons"
+ },
+ {
+ "name": "Bolowala",
+ "pos_x": 67.84375,
+ "pos_y": -58.34375,
+ "pos_z": -88.5,
+ "stations": "Stillman Terminal,Pawelczyk Enterprise,Ore Gateway"
+ },
+ {
+ "name": "Bolowari",
+ "pos_x": 27.15625,
+ "pos_y": -159.4375,
+ "pos_z": -13.34375,
+ "stations": "Robert Colony,Rizvi Station,Mil Station,Stefansson Depot"
+ },
+ {
+ "name": "Boman",
+ "pos_x": 200.53125,
+ "pos_y": -153.3125,
+ "pos_z": 89.78125,
+ "stations": "Homer Camp,Bainbridge Barracks"
+ },
+ {
+ "name": "Bomazi",
+ "pos_x": -98.75,
+ "pos_y": -20.65625,
+ "pos_z": -81.21875,
+ "stations": "Farrer Orbital,Hauck Platform,Evangelisti Beacon,Betancourt Point"
+ },
+ {
+ "name": "Bommatsuri",
+ "pos_x": 10.03125,
+ "pos_y": -104.3125,
+ "pos_z": -55.53125,
+ "stations": "Austin Market"
+ },
+ {
+ "name": "Bommegirani",
+ "pos_x": -141.09375,
+ "pos_y": 22.65625,
+ "pos_z": 80.65625,
+ "stations": "Gernhardt Base,Flade Port"
+ },
+ {
+ "name": "Bonawariyac",
+ "pos_x": 113.90625,
+ "pos_y": -91.875,
+ "pos_z": -91.59375,
+ "stations": "Watson Station,Corte-Real Relay,Robinson Horizons"
+ },
+ {
+ "name": "Bonawen",
+ "pos_x": 0.09375,
+ "pos_y": -19.53125,
+ "pos_z": 151.40625,
+ "stations": "Pordenone Port,Mohri Survey"
+ },
+ {
+ "name": "Bonde",
+ "pos_x": -17.4375,
+ "pos_y": -12.90625,
+ "pos_z": -59.375,
+ "stations": "Aksyonov Platform"
+ },
+ {
+ "name": "Bondhr",
+ "pos_x": 56.15625,
+ "pos_y": 101.5,
+ "pos_z": -28.9375,
+ "stations": "Jakes Orbital,Russ Observatory"
+ },
+ {
+ "name": "Bondranses",
+ "pos_x": -37.6875,
+ "pos_y": -12.6875,
+ "pos_z": -101.28125,
+ "stations": "Qureshi City,Monge Vision"
+ },
+ {
+ "name": "Bongk",
+ "pos_x": 160.53125,
+ "pos_y": -159.59375,
+ "pos_z": -22.53125,
+ "stations": "Zel'dovich Ring,Greenstein Installation,Dowie Port"
+ },
+ {
+ "name": "Bonitou",
+ "pos_x": -43.75,
+ "pos_y": 18.5,
+ "pos_z": -34.25,
+ "stations": "Eckford Terminal,Lyakhov City,Hughes-Fulford Terminal,Okorafor Point,Emshwiller's Folly,Menzies Depot,Teng Enterprise,Abernathy Reach"
+ },
+ {
+ "name": "Borai",
+ "pos_x": 8.09375,
+ "pos_y": -122.375,
+ "pos_z": 47.75,
+ "stations": "Wilhelm von Struve Depot,Melia Settlement,Hertzsprung Port"
+ },
+ {
+ "name": "Borann",
+ "pos_x": 123.03125,
+ "pos_y": -0.25,
+ "pos_z": 2.84375,
+ "stations": "Brothers Installation,Garay Works,Sullivan Terminal"
+ },
+ {
+ "name": "Borasetani",
+ "pos_x": 0.21875,
+ "pos_y": 117.75,
+ "pos_z": -18.03125,
+ "stations": "Katzenstein Terminal,Bulmer Depot,Hodgson Vision,Szentmartony Horizons"
+ },
+ {
+ "name": "Boreas",
+ "pos_x": 2.9375,
+ "pos_y": 64.96875,
+ "pos_z": -7.65625,
+ "stations": "Rice Gateway,Tayler Laboratory,Allen Orbital,Selberg Station,Windt Forum"
+ },
+ {
+ "name": "Boren",
+ "pos_x": 29.6875,
+ "pos_y": -149.21875,
+ "pos_z": -55.28125,
+ "stations": "Hanke-Woods' Folly,Hamuy Station,Giraud Station"
+ },
+ {
+ "name": "Boreo",
+ "pos_x": -144.25,
+ "pos_y": 14.375,
+ "pos_z": 116.28125,
+ "stations": "Peano Beacon,Gerrold Outpost"
+ },
+ {
+ "name": "Boresti",
+ "pos_x": 36.0625,
+ "pos_y": -223.25,
+ "pos_z": -39.40625,
+ "stations": "Gloss Hub,Baracchi Port"
+ },
+ {
+ "name": "Borestipi",
+ "pos_x": -161.8125,
+ "pos_y": -59.84375,
+ "pos_z": 0.96875,
+ "stations": "Sharipov Base,Shaara Keep"
+ },
+ {
+ "name": "Borfor",
+ "pos_x": -1.09375,
+ "pos_y": 21.21875,
+ "pos_z": 85.25,
+ "stations": "Al Saud Ring"
+ },
+ {
+ "name": "Bormburrun",
+ "pos_x": 57.65625,
+ "pos_y": -126.65625,
+ "pos_z": 126.03125,
+ "stations": "Schmidt Mines,Yuzhe Port,Dowling Bastion"
+ },
+ {
+ "name": "Borme",
+ "pos_x": 135.53125,
+ "pos_y": 65.375,
+ "pos_z": 122.5,
+ "stations": "Kiernan Port,Merle's Folly,McIntyre Ring,Garcia Terminal,McCarthy Keep"
+ },
+ {
+ "name": "Bormo",
+ "pos_x": 51.59375,
+ "pos_y": 2.34375,
+ "pos_z": 117.5625,
+ "stations": "Bergerac Settlement,Serre Installation,Beatty Colony"
+ },
+ {
+ "name": "Bormuninus",
+ "pos_x": -163.6875,
+ "pos_y": -51.65625,
+ "pos_z": -12.3125,
+ "stations": "Papin Port,Yamazaki Terminal,Bernoulli Terminal,Ledyard Depot"
+ },
+ {
+ "name": "Borongoreng",
+ "pos_x": -65.5,
+ "pos_y": -74.6875,
+ "pos_z": 147.1875,
+ "stations": "Lobachevsky Base"
+ },
+ {
+ "name": "Borora",
+ "pos_x": -0.75,
+ "pos_y": -71.78125,
+ "pos_z": 133.34375,
+ "stations": "May Hub,Williams Plant,Franklin's Folly"
+ },
+ {
+ "name": "Boroten",
+ "pos_x": -144.75,
+ "pos_y": -19,
+ "pos_z": -67.84375,
+ "stations": "Ordway Penal colony"
+ },
+ {
+ "name": "Borotrimpo",
+ "pos_x": -130.6875,
+ "pos_y": 0.40625,
+ "pos_z": 4.34375,
+ "stations": "Terry Platform,Pashin Outpost,Schmidt's Progress"
+ },
+ {
+ "name": "Borovii",
+ "pos_x": 76.3125,
+ "pos_y": -41.75,
+ "pos_z": -73.5625,
+ "stations": "Wrangel Reach,Bushkov Dock"
+ },
+ {
+ "name": "Borr",
+ "pos_x": 7.65625,
+ "pos_y": -31.0625,
+ "pos_z": -39.28125,
+ "stations": "Armstrong City,Grover Terminal,Howard Depot,Garriott Orbital,Kozin Prospect,Baudin Installation,Dirac Terminal,Currie City"
+ },
+ {
+ "name": "Bosagei",
+ "pos_x": -90.6875,
+ "pos_y": 46.5625,
+ "pos_z": -152.90625,
+ "stations": "Linge Platform"
+ },
+ {
+ "name": "Bosanolu",
+ "pos_x": 169.53125,
+ "pos_y": -62.34375,
+ "pos_z": 15.125,
+ "stations": "Mooz Vision"
+ },
+ {
+ "name": "Bosanos",
+ "pos_x": -58.6875,
+ "pos_y": -29.40625,
+ "pos_z": 141.59375,
+ "stations": "Ziemianski Hub,Schrodinger Lab"
+ },
+ {
+ "name": "Bosnjani",
+ "pos_x": 104.75,
+ "pos_y": 67.59375,
+ "pos_z": -0.59375,
+ "stations": "Ramelli Colony,Haignere Plant,Levy's Inheritance"
+ },
+ {
+ "name": "Bot",
+ "pos_x": -22.84375,
+ "pos_y": -52.9375,
+ "pos_z": 151.125,
+ "stations": "Usachov Port,Bobko Settlement"
+ },
+ {
+ "name": "Bota Ili",
+ "pos_x": 3.71875,
+ "pos_y": -6.78125,
+ "pos_z": -81.0625,
+ "stations": "Ashby Dock,Lister Colony"
+ },
+ {
+ "name": "Botaiti",
+ "pos_x": 111.03125,
+ "pos_y": -143.6875,
+ "pos_z": -6.53125,
+ "stations": "de Kamp Port,Stein Terminal,Humason Base,Eskridge Base,Goldreich Silo"
+ },
+ {
+ "name": "Botame",
+ "pos_x": 93.1875,
+ "pos_y": -136.15625,
+ "pos_z": -68.65625,
+ "stations": "Gora City,Sudworth Hub,Israel Station,Hume Vision,Wright Keep"
+ },
+ {
+ "name": "Boveltibes",
+ "pos_x": -7.25,
+ "pos_y": 49.9375,
+ "pos_z": 109.625,
+ "stations": "Perrin Port,Levchenko Station,Lebedev Vision,Seddon Terminal,Harris Gateway,Bertin Prospect,Aguirre Vision,Trinh Installation"
+ },
+ {
+ "name": "Boverda",
+ "pos_x": 107.0625,
+ "pos_y": 37.34375,
+ "pos_z": -16.4375,
+ "stations": "Wilson Dock,Whitson Keep"
+ },
+ {
+ "name": "BPM 16204",
+ "pos_x": 65.9375,
+ "pos_y": -161.5,
+ "pos_z": 46.75,
+ "stations": "Belyavsky Horizons,Fabian Mines"
+ },
+ {
+ "name": "BPM 28514",
+ "pos_x": 47.09375,
+ "pos_y": -114.34375,
+ "pos_z": 48.75,
+ "stations": "Salam Survey,Wood Horizons,Farrukh Camp"
+ },
+ {
+ "name": "BPM 40823",
+ "pos_x": 30.875,
+ "pos_y": -8,
+ "pos_z": 109.71875,
+ "stations": "Konscak Survey,Thomas' Claim"
+ },
+ {
+ "name": "BPM 45047",
+ "pos_x": 15.28125,
+ "pos_y": -67.84375,
+ "pos_z": 28.375,
+ "stations": "Thompson City,Wells Beacon,Fraley Orbital,Gagnan Orbital,Marshall Beacon,Phillpotts Palace"
+ },
+ {
+ "name": "BPM 590",
+ "pos_x": -2.40625,
+ "pos_y": -105.5625,
+ "pos_z": 0.6875,
+ "stations": "Foster Vision"
+ },
+ {
+ "name": "BPM 62891",
+ "pos_x": -14.34375,
+ "pos_y": 1.3125,
+ "pos_z": 102.71875,
+ "stations": "Zhigang Platform,Akers Installation"
+ },
+ {
+ "name": "BPM 72121",
+ "pos_x": 78.21875,
+ "pos_y": -15.78125,
+ "pos_z": -67.6875,
+ "stations": "Cori Port,Kinsey Dock,Minkowski Relay"
+ },
+ {
+ "name": "BPM 87242",
+ "pos_x": 19.59375,
+ "pos_y": 103.5,
+ "pos_z": -33.625,
+ "stations": "Smith Station"
+ },
+ {
+ "name": "BPM 89444",
+ "pos_x": -0.90625,
+ "pos_y": 84.5625,
+ "pos_z": 31.90625,
+ "stations": "Rosenberg Station,Szameit Ring,al-Haytham Port,Quinn Terminal,Oltion Barracks"
+ },
+ {
+ "name": "BR Piscium",
+ "pos_x": -10.625,
+ "pos_y": -16.34375,
+ "pos_z": -0.90625,
+ "stations": "Trevithick Orbital,Cabrera Point"
+ },
+ {
+ "name": "Brabara",
+ "pos_x": -81.78125,
+ "pos_y": -11.65625,
+ "pos_z": 144.875,
+ "stations": "Jendrassik Point,Detmer Dock,Doi Base"
+ },
+ {
+ "name": "Brabralla",
+ "pos_x": 114.875,
+ "pos_y": 75.75,
+ "pos_z": -68.125,
+ "stations": "Low Platform,Laplace Beacon"
+ },
+ {
+ "name": "Brabralung",
+ "pos_x": 96.84375,
+ "pos_y": -45.8125,
+ "pos_z": 132.9375,
+ "stations": "Riazuddin Settlement,Rogers Platform"
+ },
+ {
+ "name": "Brabri",
+ "pos_x": -127.6875,
+ "pos_y": 32.09375,
+ "pos_z": 1.40625,
+ "stations": "Eilenberg Beacon,von Krusenstern Port"
+ },
+ {
+ "name": "Bracari",
+ "pos_x": -132.4375,
+ "pos_y": -43.46875,
+ "pos_z": -80.46875,
+ "stations": "Langsdorff Mines"
+ },
+ {
+ "name": "Bragande",
+ "pos_x": 106.09375,
+ "pos_y": -180.875,
+ "pos_z": -50.1875,
+ "stations": "Bailly Mines,Horrocks Port,Chun's Progress,Boss Vision"
+ },
+ {
+ "name": "Bragarijin",
+ "pos_x": 14.78125,
+ "pos_y": -44.40625,
+ "pos_z": 128.34375,
+ "stations": "Lee Depot,Phillips Settlement,Gunn Bastion"
+ },
+ {
+ "name": "Bragit",
+ "pos_x": 143.1875,
+ "pos_y": -129.40625,
+ "pos_z": -61.8125,
+ "stations": "Tousey Terminal,Opik Hub,Brorsen Port,Grant's Inheritance"
+ },
+ {
+ "name": "Bragpura",
+ "pos_x": -81.5,
+ "pos_y": -3.875,
+ "pos_z": -77.875,
+ "stations": "Eyharts Plant,Halsell Enterprise,Sturckow Landing"
+ },
+ {
+ "name": "Bragpurukab",
+ "pos_x": -21.28125,
+ "pos_y": -22.9375,
+ "pos_z": 97.71875,
+ "stations": "Zalyotin Hub"
+ },
+ {
+ "name": "Bragur",
+ "pos_x": 94.03125,
+ "pos_y": -113.125,
+ "pos_z": -5.25,
+ "stations": "Bulychev Enterprise,Altshuller Terminal,Christian Station,Sekelj Bastion,Salgari Station,White Enterprise,Neville Port,Wood Enterprise,Szentmartony Enterprise,Filter Terminal,Gora Escape,Abbott's Claim"
+ },
+ {
+ "name": "Bragurom Du",
+ "pos_x": 17.4375,
+ "pos_y": 61.1875,
+ "pos_z": -6.53125,
+ "stations": "Pellegrino Station,Barnaby Gateway"
+ },
+ {
+ "name": "Brahma",
+ "pos_x": 13.28125,
+ "pos_y": 45.25,
+ "pos_z": 17.65625,
+ "stations": "Jenner Terminal,Sharipov Hub,Rae Legacy,Hughes Depot,Smith Terminal"
+ },
+ {
+ "name": "Brahman",
+ "pos_x": 136.34375,
+ "pos_y": -117.96875,
+ "pos_z": 82.125,
+ "stations": "Frost Base,Fox Colony"
+ },
+ {
+ "name": "Braman",
+ "pos_x": 88.03125,
+ "pos_y": -65.0625,
+ "pos_z": 22,
+ "stations": "Pinzon's Exile,Barr's Inheritance"
+ },
+ {
+ "name": "Branara",
+ "pos_x": 100.375,
+ "pos_y": 69.125,
+ "pos_z": -68.625,
+ "stations": "Plante Prospect,Marlowe's Folly"
+ },
+ {
+ "name": "Brandano",
+ "pos_x": 64.84375,
+ "pos_y": -29.03125,
+ "pos_z": 38.125,
+ "stations": "Parise Landing,Lenoir Depot"
+ },
+ {
+ "name": "Brangkalar",
+ "pos_x": -92.78125,
+ "pos_y": -34.9375,
+ "pos_z": -63.8125,
+ "stations": "Jeury Landing"
+ },
+ {
+ "name": "Branglal",
+ "pos_x": 18.90625,
+ "pos_y": 34.28125,
+ "pos_z": -77.15625,
+ "stations": "RenenBellot Relay,Skripochka Gateway,Gibson Horizons,Cole Keep"
+ },
+ {
+ "name": "Brani",
+ "pos_x": -65.90625,
+ "pos_y": -11.8125,
+ "pos_z": 52.53125,
+ "stations": "Hooke Enterprise,Virtanen Hub,Wundt Hub,Barsanti Enterprise,Francisco de Eliza Port,Flade Palace,Akiyama Market,Hadfield Port,Noakes Port,Papin Hub,Goulart Horizons"
+ },
+ {
+ "name": "Brata",
+ "pos_x": -42.3125,
+ "pos_y": -100.90625,
+ "pos_z": 96.65625,
+ "stations": "Leonard Terminal,Grigson Relay"
+ },
+ {
+ "name": "Bratabha",
+ "pos_x": 56.59375,
+ "pos_y": -170.3125,
+ "pos_z": -30.0625,
+ "stations": "Kobayashi Vision,Hughes Terminal,Perga's Inheritance,Dalgarno Orbital,Brooks Vision"
+ },
+ {
+ "name": "Bratadi",
+ "pos_x": 202.90625,
+ "pos_y": -146.75,
+ "pos_z": 89.78125,
+ "stations": "Grunsfeld Dock"
+ },
+ {
+ "name": "Bratauolung",
+ "pos_x": -122.15625,
+ "pos_y": -69.46875,
+ "pos_z": 54.34375,
+ "stations": "Lee Colony,Ford Bastion,Griffiths Camp,Roggeveen Colony"
+ },
+ {
+ "name": "Bratio",
+ "pos_x": -61.71875,
+ "pos_y": -162.84375,
+ "pos_z": -78.96875,
+ "stations": "Morgan Dock,Ross Settlement,Galouye Landing,Whymper Colony"
+ },
+ {
+ "name": "Bratisas",
+ "pos_x": -70.9375,
+ "pos_y": -80.125,
+ "pos_z": -122.5,
+ "stations": "Atwater Station,la Cosa Keep,Nicollier Plant"
+ },
+ {
+ "name": "Breamen",
+ "pos_x": -79.46875,
+ "pos_y": -142.21875,
+ "pos_z": -11.71875,
+ "stations": "Allat39,Velho Dock,Quick Works"
+ },
+ {
+ "name": "Breksta",
+ "pos_x": 29.4375,
+ "pos_y": -6.71875,
+ "pos_z": -70.46875,
+ "stations": "Barcelos City,Legendre Ring,Perez Market,Narvaez Orbital,Wells Hub,Knight Survey,Eudoxus Installation,Pierres Ring,Popper Dock,Grant Terminal,Doctorow Installation,Brooks City"
+ },
+ {
+ "name": "Bresatese",
+ "pos_x": -9.0625,
+ "pos_y": 15.25,
+ "pos_z": 119.1875,
+ "stations": "de Andrade Terminal,Solovyov Colony,Ejeta Landing"
+ },
+ {
+ "name": "Brestla",
+ "pos_x": 159.46875,
+ "pos_y": -103.75,
+ "pos_z": -19.6875,
+ "stations": "Roed Odegaard Port,i Sola Prospect,Weaver Refinery,Powers Horizons,Faye Mines"
+ },
+ {
+ "name": "Breucosia",
+ "pos_x": 81.09375,
+ "pos_y": 32.59375,
+ "pos_z": 117.59375,
+ "stations": "Blodgett Enterprise,Thornton Platform,al-Din Terminal"
+ },
+ {
+ "name": "Breucosyen",
+ "pos_x": -164.65625,
+ "pos_y": 59.28125,
+ "pos_z": -5,
+ "stations": "Due Landing"
+ },
+ {
+ "name": "Brian",
+ "pos_x": 22.9375,
+ "pos_y": -226.125,
+ "pos_z": 23.34375,
+ "stations": "Geller Platform,Cuffey Vision,Fanning Horizons"
+ },
+ {
+ "name": "Brians",
+ "pos_x": 107.75,
+ "pos_y": -135.15625,
+ "pos_z": -8.40625,
+ "stations": "Kuo Hub,Kimura Vision,Busemann Port,Zwicky Station,Newcomb Gateway,Ball Vision,Morrill Dock,Hirn Base,Galindo Point,Ilyushin Orbital,Scotti Holdings,Heiles City,Kojima Arsenal"
+ },
+ {
+ "name": "Brib",
+ "pos_x": 90.65625,
+ "pos_y": -106.5,
+ "pos_z": 11.25,
+ "stations": "Dominique Holdings,Ludwig Struve Landing,Kludze Landing"
+ },
+ {
+ "name": "Bribroingga",
+ "pos_x": -45.40625,
+ "pos_y": -155.375,
+ "pos_z": 80.84375,
+ "stations": "Baynes Dock,Williams Lab"
+ },
+ {
+ "name": "Brid",
+ "pos_x": -118.25,
+ "pos_y": 33.34375,
+ "pos_z": -23.65625,
+ "stations": "Salgari Platform,Weierstrass Prospect,Beaufoy's Folly"
+ },
+ {
+ "name": "Bridge",
+ "pos_x": -83.4375,
+ "pos_y": -100.21875,
+ "pos_z": 95.0625,
+ "stations": "Caselberg Relay,Kornbluth Prospect,Coelho Dock"
+ },
+ {
+ "name": "Bridi",
+ "pos_x": 102.125,
+ "pos_y": -73.40625,
+ "pos_z": 26.875,
+ "stations": "Harawi Vision,Borrelly Port,Sudworth Gateway,Ziemkiewicz Landing"
+ },
+ {
+ "name": "Brigae",
+ "pos_x": -22.03125,
+ "pos_y": -159.65625,
+ "pos_z": 68.96875,
+ "stations": "Chiang Colony,Lanier Laboratory"
+ },
+ {
+ "name": "Brigaentini",
+ "pos_x": 171.0625,
+ "pos_y": -10.375,
+ "pos_z": -29.46875,
+ "stations": "Andree Installation,Maler Freeport"
+ },
+ {
+ "name": "Brigama",
+ "pos_x": 90.75,
+ "pos_y": -168.59375,
+ "pos_z": 166.28125,
+ "stations": "Mrkos Platform,Kelly Platform,Ackerman Horizons"
+ },
+ {
+ "name": "Brigan",
+ "pos_x": 160.40625,
+ "pos_y": -39.3125,
+ "pos_z": -38.84375,
+ "stations": "Bethke Beacon"
+ },
+ {
+ "name": "Briganii",
+ "pos_x": -90.375,
+ "pos_y": 133.65625,
+ "pos_z": 0.15625,
+ "stations": "Kingsmill Ring,Napier Hub,Hambly Colony"
+ },
+ {
+ "name": "Briganu",
+ "pos_x": 88.71875,
+ "pos_y": -31.03125,
+ "pos_z": 16.53125,
+ "stations": "Vinogradov Stop"
+ },
+ {
+ "name": "Brigh",
+ "pos_x": 149.96875,
+ "pos_y": -9.625,
+ "pos_z": 9.0625,
+ "stations": "Reightler Base,Weierstrass Orbital"
+ },
+ {
+ "name": "Brigid",
+ "pos_x": -11.125,
+ "pos_y": -164.5,
+ "pos_z": 19.21875,
+ "stations": "Unsold City,Altuna Vision,Sagan Depot,Orban Port"
+ },
+ {
+ "name": "Brigua",
+ "pos_x": -159.15625,
+ "pos_y": -69.75,
+ "pos_z": -35.40625,
+ "stations": "Navigator Outpost"
+ },
+ {
+ "name": "Brigual",
+ "pos_x": 33.25,
+ "pos_y": -214.3125,
+ "pos_z": -39.25,
+ "stations": "Sudworth Port"
+ },
+ {
+ "name": "Brigus",
+ "pos_x": -11.78125,
+ "pos_y": -200,
+ "pos_z": 39.84375,
+ "stations": "Tanaka Hub,Palmer Installation,Kolmogorov Enterprise"
+ },
+ {
+ "name": "Brigusia",
+ "pos_x": 167.15625,
+ "pos_y": -74.625,
+ "pos_z": 66.40625,
+ "stations": "Tarter Vision,Riebe Survey,Cheranovsky Works"
+ },
+ {
+ "name": "Brihasatsa",
+ "pos_x": 36.40625,
+ "pos_y": -54.03125,
+ "pos_z": -55.25,
+ "stations": "Howe Enterprise,Chaudhary Port,Pythagoras Enterprise,Cramer Point"
+ },
+ {
+ "name": "Brihaspati",
+ "pos_x": 140.21875,
+ "pos_y": 54.46875,
+ "pos_z": 34.40625,
+ "stations": "Fowler Relay,Divis Installation"
+ },
+ {
+ "name": "Brivitt",
+ "pos_x": -45.8125,
+ "pos_y": -63.09375,
+ "pos_z": -36.875,
+ "stations": "Parsons Terminal,Georg Bothe City"
+ },
+ {
+ "name": "Brizo",
+ "pos_x": -20.21875,
+ "pos_y": 71.65625,
+ "pos_z": -29.15625,
+ "stations": "Crowley Barracks,Resnick Platform"
+ },
+ {
+ "name": "Brodal",
+ "pos_x": 40.9375,
+ "pos_y": 67.6875,
+ "pos_z": -33.46875,
+ "stations": "Guerrero Survey,Besonders Landing,Budrys Landing"
+ },
+ {
+ "name": "Brohman",
+ "pos_x": 142.03125,
+ "pos_y": -25.875,
+ "pos_z": 49.90625,
+ "stations": "Filipchenko Installation,Pontes Legacy"
+ },
+ {
+ "name": "Brokpa",
+ "pos_x": 129,
+ "pos_y": -62.71875,
+ "pos_z": 64.71875,
+ "stations": "Giancola Holdings,Lane Colony,Kimura Terminal"
+ },
+ {
+ "name": "Brokpoi",
+ "pos_x": -1.09375,
+ "pos_y": -4.4375,
+ "pos_z": 150.9375,
+ "stations": "Williams Platform,Reed Settlement,Anderson Barracks"
+ },
+ {
+ "name": "Brokutsu",
+ "pos_x": -57.21875,
+ "pos_y": 11.6875,
+ "pos_z": -31.9375,
+ "stations": "Shuttleworth Colony,Sturt Prospect,Grandin Hub"
+ },
+ {
+ "name": "Brontes",
+ "pos_x": 8.6875,
+ "pos_y": 42.46875,
+ "pos_z": -45.125,
+ "stations": "Thorne Hub"
+ },
+ {
+ "name": "Brulao",
+ "pos_x": 77.28125,
+ "pos_y": 43.78125,
+ "pos_z": -64.84375,
+ "stations": "Brady Dock"
+ },
+ {
+ "name": "Brulebegera",
+ "pos_x": 165.59375,
+ "pos_y": -101.03125,
+ "pos_z": 91.9375,
+ "stations": "Paola Beacon,McCrea Dock"
+ },
+ {
+ "name": "Brulenjan",
+ "pos_x": 22.0625,
+ "pos_y": 70.09375,
+ "pos_z": 81.65625,
+ "stations": "Brooks Prospect"
+ },
+ {
+ "name": "Bruli",
+ "pos_x": -126.78125,
+ "pos_y": 59.53125,
+ "pos_z": 75.46875,
+ "stations": "Shaikh Plant,Skolem Platform"
+ },
+ {
+ "name": "Brulidji",
+ "pos_x": 48.90625,
+ "pos_y": -212.71875,
+ "pos_z": 50.46875,
+ "stations": "Urata Hub,Townes Hub,Montanari Port,Hedin Vision,Bowell Installation"
+ },
+ {
+ "name": "Brultamaci",
+ "pos_x": -153.8125,
+ "pos_y": -74.46875,
+ "pos_z": 20.34375,
+ "stations": "Pippin Dock,Herjulfsson Dock,Shiras Prospect,Celebi Bastion"
+ },
+ {
+ "name": "Brunne",
+ "pos_x": 30.125,
+ "pos_y": -42.125,
+ "pos_z": -68.90625,
+ "stations": "Tesla Station,Taylor Installation"
+ },
+ {
+ "name": "Brutas",
+ "pos_x": -60.71875,
+ "pos_y": 32.59375,
+ "pos_z": 91.90625,
+ "stations": "Comer Dock,Collins Dock"
+ },
+ {
+ "name": "Bruth",
+ "pos_x": 116.0625,
+ "pos_y": -38.125,
+ "pos_z": -21.71875,
+ "stations": "Bombelli Terminal,Laird Dock,Siegel Arena"
+ },
+ {
+ "name": "Bruthanvan",
+ "pos_x": 80.65625,
+ "pos_y": 42.28125,
+ "pos_z": 57.84375,
+ "stations": "Lovell Depot"
+ },
+ {
+ "name": "Brynhilo",
+ "pos_x": -122.0625,
+ "pos_y": -12,
+ "pos_z": 39.71875,
+ "stations": "Manarov Orbital"
+ },
+ {
+ "name": "Brynicnal",
+ "pos_x": 50.21875,
+ "pos_y": 97.625,
+ "pos_z": -111.46875,
+ "stations": "Read's Claim"
+ },
+ {
+ "name": "BU 741",
+ "pos_x": 20.5,
+ "pos_y": -64.21875,
+ "pos_z": -28.75,
+ "stations": "Starport Alpha"
+ },
+ {
+ "name": "Buan Kuan",
+ "pos_x": -87.03125,
+ "pos_y": 126,
+ "pos_z": -32.75,
+ "stations": "Elgin Prospect,Garden Settlement,Hinz Colony"
+ },
+ {
+ "name": "Buan Zha",
+ "pos_x": 90,
+ "pos_y": -30.90625,
+ "pos_z": 87.3125,
+ "stations": "Oluwafemi Settlement,Bosch Depot,Adams Vision"
+ },
+ {
+ "name": "Bubians",
+ "pos_x": -6.5,
+ "pos_y": 12.9375,
+ "pos_z": -137.71875,
+ "stations": "Reed Outpost"
+ },
+ {
+ "name": "Bubii",
+ "pos_x": 111.5,
+ "pos_y": -204.03125,
+ "pos_z": -61.125,
+ "stations": "Cerulli Survey,Zhuravleva Dock,Campbell Terminal"
+ },
+ {
+ "name": "Bugaga",
+ "pos_x": -96.59375,
+ "pos_y": 28.21875,
+ "pos_z": 112.96875,
+ "stations": "Kelly City,Vetulani Dock,Artin Depot"
+ },
+ {
+ "name": "Bugaguti",
+ "pos_x": 150.96875,
+ "pos_y": -200.09375,
+ "pos_z": 103.375,
+ "stations": "Fox's Claim"
+ },
+ {
+ "name": "Buganda",
+ "pos_x": 166.65625,
+ "pos_y": -51.53125,
+ "pos_z": -18.25,
+ "stations": "Farghani Enterprise,Mizuno Port,Fife Landing"
+ },
+ {
+ "name": "Bugari",
+ "pos_x": -166.1875,
+ "pos_y": -2.34375,
+ "pos_z": 99.375,
+ "stations": "Fibonacci Settlement,Bradbury Terminal,Bulgarin Beacon"
+ },
+ {
+ "name": "Bugas",
+ "pos_x": 12.59375,
+ "pos_y": 24.34375,
+ "pos_z": 2.84375,
+ "stations": "Lander Vision,Friend Terminal,Disch Station,Marlowe Orbital,Oliver Ring,Oliver Dock,Burstein Terminal,Wilhelm Terminal,Malchiodi Dock,Fawcett Landing,Belyanin Hub,Ledyard Enterprise"
+ },
+ {
+ "name": "Bugayaman",
+ "pos_x": 55.0625,
+ "pos_y": 87.28125,
+ "pos_z": 56.78125,
+ "stations": "Asprin Orbital"
+ },
+ {
+ "name": "Bugua",
+ "pos_x": -63.125,
+ "pos_y": -62.78125,
+ "pos_z": -122.59375,
+ "stations": "Gauss Vision"
+ },
+ {
+ "name": "Bugulmara",
+ "pos_x": 74.34375,
+ "pos_y": 111.15625,
+ "pos_z": 114.90625,
+ "stations": "Bruce Port,Coulomb Port,Malcolm Colony,Baraniecki Installation"
+ },
+ {
+ "name": "Buku Mana",
+ "pos_x": 160.15625,
+ "pos_y": -84.6875,
+ "pos_z": -22.65625,
+ "stations": "Moffat Landing,Jean Platform,Smirnova Settlement"
+ },
+ {
+ "name": "Bukua",
+ "pos_x": -46.5625,
+ "pos_y": -131.96875,
+ "pos_z": -53.4375,
+ "stations": "Harvey Orbital,Volynov Refinery,Issigonis Orbital,Leestma Ring,Carstensz Silo"
+ },
+ {
+ "name": "Bukulas",
+ "pos_x": -44,
+ "pos_y": -71.96875,
+ "pos_z": -127.375,
+ "stations": "Trevithick Station,Poindexter Beacon,Fermat Installation"
+ },
+ {
+ "name": "Bukurnabal",
+ "pos_x": -46.5625,
+ "pos_y": 100.78125,
+ "pos_z": 32.5625,
+ "stations": "Kneale Terminal"
+ },
+ {
+ "name": "Bulganjoba",
+ "pos_x": 49.96875,
+ "pos_y": -180.84375,
+ "pos_z": 54.21875,
+ "stations": "Dunlop Relay,William Sargent Mines,Stebbins Settlement"
+ },
+ {
+ "name": "Bulgarlmadi",
+ "pos_x": 130.5625,
+ "pos_y": -108.15625,
+ "pos_z": 48.875,
+ "stations": "Hoffmeister City,Eggleton Terminal,Dittmar Orbital,Arp Base"
+ },
+ {
+ "name": "Bulkabil",
+ "pos_x": 161.84375,
+ "pos_y": -123.40625,
+ "pos_z": 105.9375,
+ "stations": "Jansky Station,Kepler Installation,Riess Horizons,Tanaka Vision,Gwynn Depot"
+ },
+ {
+ "name": "Bulku",
+ "pos_x": 72.5,
+ "pos_y": -38.84375,
+ "pos_z": -48.40625,
+ "stations": "Reilly Base,Dobzhansky Settlement"
+ },
+ {
+ "name": "Bulkun",
+ "pos_x": 24.4375,
+ "pos_y": -138.90625,
+ "pos_z": 149.875,
+ "stations": "Kopff Works"
+ },
+ {
+ "name": "Bulkuq",
+ "pos_x": 143.125,
+ "pos_y": -87.3125,
+ "pos_z": -7.78125,
+ "stations": "Smoot Station"
+ },
+ {
+ "name": "Bulkuylkana",
+ "pos_x": 135.90625,
+ "pos_y": 10.8125,
+ "pos_z": 20.5625,
+ "stations": "Shiras Dock,Tokubei Dock,Bolton Plant,Sladek Dock,Gunn's Progress"
+ },
+ {
+ "name": "Bullpop",
+ "pos_x": -36.15625,
+ "pos_y": 54.6875,
+ "pos_z": 58.59375,
+ "stations": "Kooi Horizons,Vasyutin Platform,Al Saud Depot"
+ },
+ {
+ "name": "Bulun",
+ "pos_x": -44.59375,
+ "pos_y": -132.65625,
+ "pos_z": 118,
+ "stations": "Thurston's Inheritance"
+ },
+ {
+ "name": "Bulungu",
+ "pos_x": 33.71875,
+ "pos_y": -112.90625,
+ "pos_z": -53.375,
+ "stations": "Evans Colony,Hariot Vision"
+ },
+ {
+ "name": "Buluujja",
+ "pos_x": 119.15625,
+ "pos_y": -59.53125,
+ "pos_z": -70.9375,
+ "stations": "Littrow Stop,von Bellingshausen Relay"
+ },
+ {
+ "name": "Buluwai",
+ "pos_x": 10.53125,
+ "pos_y": -28.40625,
+ "pos_z": 111.78125,
+ "stations": "Chebyshev Gateway"
+ },
+ {
+ "name": "Bumba",
+ "pos_x": -88.28125,
+ "pos_y": 59.65625,
+ "pos_z": -92.4375,
+ "stations": "Lavoisier Hangar"
+ },
+ {
+ "name": "Bumbindal",
+ "pos_x": 84.1875,
+ "pos_y": -166.15625,
+ "pos_z": 140.0625,
+ "stations": "Watson Port,Harding Vision,Ludwig Struve Orbital,Korolev Silo"
+ },
+ {
+ "name": "Bumbo",
+ "pos_x": -92.0625,
+ "pos_y": 35,
+ "pos_z": -64.28125,
+ "stations": "Hooke Relay,Watt Mine"
+ },
+ {
+ "name": "Bumbojas",
+ "pos_x": -31.53125,
+ "pos_y": -183.4375,
+ "pos_z": -51.71875,
+ "stations": "Goryu Arena,Dalgarno Survey"
+ },
+ {
+ "name": "Bumbur",
+ "pos_x": -87.375,
+ "pos_y": -3.75,
+ "pos_z": -45.84375,
+ "stations": "Lundwall Dock,Tolstoi Landing,Lee Landing"
+ },
+ {
+ "name": "Bunbudo",
+ "pos_x": 65.71875,
+ "pos_y": -260.84375,
+ "pos_z": -7.4375,
+ "stations": "Artzybasheff Port,van Vogt Arsenal"
+ },
+ {
+ "name": "Bunbulama",
+ "pos_x": 123.03125,
+ "pos_y": -226.65625,
+ "pos_z": 64.65625,
+ "stations": "Paczynski Terminal,Naboth Landing,Walotsky Base"
+ },
+ {
+ "name": "Bunda",
+ "pos_x": 65.625,
+ "pos_y": 17.96875,
+ "pos_z": 24.96875,
+ "stations": "Faraday Ring,Walz Dock,Cori Orbital"
+ },
+ {
+ "name": "Bundena",
+ "pos_x": 40.03125,
+ "pos_y": -89.34375,
+ "pos_z": -57.5625,
+ "stations": "Behnisch Hangar,Barr Terminal"
+ },
+ {
+ "name": "Bundhar",
+ "pos_x": 9.28125,
+ "pos_y": 13.65625,
+ "pos_z": -115.46875,
+ "stations": "Leibniz Depot"
+ },
+ {
+ "name": "Bunganditj",
+ "pos_x": -128.75,
+ "pos_y": -9.34375,
+ "pos_z": -48.59375,
+ "stations": "Schrodinger Vision,Nye Depot"
+ },
+ {
+ "name": "Bungk",
+ "pos_x": -60.15625,
+ "pos_y": -8.09375,
+ "pos_z": 159.71875,
+ "stations": "Legendre Port,Rolland Vision,Vonarburg Terminal"
+ },
+ {
+ "name": "Bunjil",
+ "pos_x": -56.34375,
+ "pos_y": -162.8125,
+ "pos_z": -14.75,
+ "stations": "Parsons Point"
+ },
+ {
+ "name": "Bunuras",
+ "pos_x": 56.75,
+ "pos_y": -17.90625,
+ "pos_z": -0.3125,
+ "stations": "Noguchi Enterprise,Galouye Bastion,Hilmers Lab,Franklin City"
+ },
+ {
+ "name": "Bunus",
+ "pos_x": 39.53125,
+ "pos_y": 29.9375,
+ "pos_z": 47.5,
+ "stations": "Jones Freeport,Baxter Base"
+ },
+ {
+ "name": "Bunuson",
+ "pos_x": 111.90625,
+ "pos_y": -104.90625,
+ "pos_z": -16.09375,
+ "stations": "Stackpole Survey,Burnham Ring,Adragna Arena,Arzachel Terminal,Antoniadi Installation"
+ },
+ {
+ "name": "Bunuvivia",
+ "pos_x": -104.34375,
+ "pos_y": 28.46875,
+ "pos_z": 59.34375,
+ "stations": "Greenland City,Wrangel Depot,Ellison Enterprise"
+ },
+ {
+ "name": "Bunyindjana",
+ "pos_x": -22.65625,
+ "pos_y": -22.25,
+ "pos_z": 115.25,
+ "stations": "Bernard Mine,Clervoy Station"
+ },
+ {
+ "name": "Buri",
+ "pos_x": 64.9375,
+ "pos_y": 44.84375,
+ "pos_z": -143.8125,
+ "stations": "Burnell Settlement,Eddington Terminal,Krupkat Arsenal"
+ },
+ {
+ "name": "Buricasses",
+ "pos_x": 4.3125,
+ "pos_y": -102,
+ "pos_z": 45.6875,
+ "stations": "Walotsky Gateway"
+ },
+ {
+ "name": "Buriges",
+ "pos_x": 110.34375,
+ "pos_y": -118.375,
+ "pos_z": -26.03125,
+ "stations": "Shirazi City,Rubin Orbital,Palisa Keep,Pennington Vision,Bass Vista"
+ },
+ {
+ "name": "Burigo",
+ "pos_x": 111.96875,
+ "pos_y": -52.6875,
+ "pos_z": 119.9375,
+ "stations": "Burkin Base,Aaronson's Claim"
+ },
+ {
+ "name": "Burigpa",
+ "pos_x": -84.09375,
+ "pos_y": -100.15625,
+ "pos_z": -82.40625,
+ "stations": "Wnuk-Lipinski Platform,Hawking Depot"
+ },
+ {
+ "name": "Burious",
+ "pos_x": 60.0625,
+ "pos_y": -122.96875,
+ "pos_z": 115.8125,
+ "stations": "Rittenhouse Colony,Williamson Oasis,Chernykh Dock,Behnisch Estate,Aitken Base"
+ },
+ {
+ "name": "Burod",
+ "pos_x": -16,
+ "pos_y": -197.375,
+ "pos_z": 40.59375,
+ "stations": "Dilworth Survey,Babakin Dock,Hale Prospect,Lindemann Vision"
+ },
+ {
+ "name": "Burojinura",
+ "pos_x": 17.96875,
+ "pos_y": -20.375,
+ "pos_z": 54.21875,
+ "stations": "Smith City,Griffith Station,Kier Station,Davidson Terminal,Stephens Enterprise,Merbold Works,MacVicar Depot,Madsen Settlement"
+ },
+ {
+ "name": "Burok",
+ "pos_x": 116.03125,
+ "pos_y": 20.09375,
+ "pos_z": 30.34375,
+ "stations": "Gubarev Port,Thiele Holdings"
+ },
+ {
+ "name": "Burr",
+ "pos_x": -27.96875,
+ "pos_y": 10.96875,
+ "pos_z": -89.875,
+ "stations": "Shepherd Beacon,Brunton Outpost,Cook Silo"
+ },
+ {
+ "name": "Buryang",
+ "pos_x": 118.8125,
+ "pos_y": 37.9375,
+ "pos_z": -99.625,
+ "stations": "Kelleam Dock"
+ },
+ {
+ "name": "Bushur",
+ "pos_x": 97.9375,
+ "pos_y": -239.8125,
+ "pos_z": 39.15625,
+ "stations": "Stromgren Mines"
+ },
+ {
+ "name": "Butors",
+ "pos_x": 73,
+ "pos_y": -91.5,
+ "pos_z": 60.59375,
+ "stations": "Tuttle Point,Koolhaas Platform,Babcock Vision"
+ },
+ {
+ "name": "Buyuma",
+ "pos_x": -130.625,
+ "pos_y": -23.25,
+ "pos_z": -51.3125,
+ "stations": "Tyurin City,Marshburn Platform"
+ },
+ {
+ "name": "Buzhang Ku",
+ "pos_x": 44.03125,
+ "pos_y": -52.4375,
+ "pos_z": -45.9375,
+ "stations": "Hermaszewski Hub,Coats Terminal"
+ },
+ {
+ "name": "Buzhangga",
+ "pos_x": 108.9375,
+ "pos_y": -45.96875,
+ "pos_z": 128.4375,
+ "stations": "Adragna Vision,Finlay-Freundlich Platform,Bradbury Survey"
+ },
+ {
+ "name": "BV Phoenicis",
+ "pos_x": 41.53125,
+ "pos_y": -106.09375,
+ "pos_z": 24.5625,
+ "stations": "Aoki Dock,Kahn Enterprise,Vyssotsky Orbital"
+ },
+ {
+ "name": "BW Phoenix",
+ "pos_x": 47.59375,
+ "pos_y": -120.59375,
+ "pos_z": 27.53125,
+ "stations": "Barnwell Stop"
+ },
+ {
+ "name": "Bwena",
+ "pos_x": 122.375,
+ "pos_y": -9.15625,
+ "pos_z": 80.59375,
+ "stations": "Sakers Hub,Milne Relay"
+ },
+ {
+ "name": "Bwenapok",
+ "pos_x": 72.15625,
+ "pos_y": -159.90625,
+ "pos_z": 179.8125,
+ "stations": "Hickman Port,Molyneux Point,Anthony Hub"
+ },
+ {
+ "name": "Bwgcollowah",
+ "pos_x": 134.375,
+ "pos_y": -122.1875,
+ "pos_z": 38.3125,
+ "stations": "Xuesen Platform"
+ },
+ {
+ "name": "Bwgcolmasci",
+ "pos_x": 33.34375,
+ "pos_y": -18.8125,
+ "pos_z": -156.03125,
+ "stations": "Hedin Station,Finch Enterprise,Snodgrass Ring,Judson Barracks"
+ },
+ {
+ "name": "Bwilota",
+ "pos_x": -16.59375,
+ "pos_y": -170.53125,
+ "pos_z": -39.5,
+ "stations": "Gustav Sporer Landing,Harawi Port,Brackett Vision"
+ },
+ {
+ "name": "Byel Taca",
+ "pos_x": -12.21875,
+ "pos_y": -20.0625,
+ "pos_z": -25.25,
+ "stations": "Krupkat Enterprise,Melroy Horizons"
+ },
+ {
+ "name": "Byel Tan",
+ "pos_x": -96.625,
+ "pos_y": 13.15625,
+ "pos_z": 92.1875,
+ "stations": "Wafer Terminal,Barcelo Refinery"
+ },
+ {
+ "name": "Byeri",
+ "pos_x": 18.28125,
+ "pos_y": -134.09375,
+ "pos_z": -16.15625,
+ "stations": "Windt Colony,Chaviano City,Gibson Gateway"
+ },
+ {
+ "name": "Byerri",
+ "pos_x": 155.4375,
+ "pos_y": -110.625,
+ "pos_z": 94.0625,
+ "stations": "Challis Landing,Lemaitre Dock,Burstein Enterprise"
+ },
+ {
+ "name": "Byeru Bese",
+ "pos_x": -55.84375,
+ "pos_y": -91.03125,
+ "pos_z": 45.1875,
+ "stations": "Chebyshev Dock,Garnier Station,Tall Station,Bradley Prospect,Asprin Arsenal"
+ },
+ {
+ "name": "Byerus",
+ "pos_x": -116.25,
+ "pos_y": -53.78125,
+ "pos_z": 97.59375,
+ "stations": "Maybury Bastion,Wilder Dock"
+ },
+ {
+ "name": "Byggvi",
+ "pos_x": 23.375,
+ "pos_y": 150.34375,
+ "pos_z": 37.3125,
+ "stations": "Kondakova Beacon"
+ },
+ {
+ "name": "Bylgiani",
+ "pos_x": 117.78125,
+ "pos_y": 9.78125,
+ "pos_z": 96.96875,
+ "stations": "May's Exile,Coblentz Terminal"
+ },
+ {
+ "name": "Bylgit",
+ "pos_x": -80.71875,
+ "pos_y": 78.875,
+ "pos_z": -41.59375,
+ "stations": "Thornycroft Gateway,Harrison City,Sinclair Silo"
+ },
+ {
+ "name": "Bylli",
+ "pos_x": 161.75,
+ "pos_y": -66.46875,
+ "pos_z": 31.25,
+ "stations": "Burnham Stop"
+ },
+ {
+ "name": "Byllia",
+ "pos_x": 119.125,
+ "pos_y": -100.375,
+ "pos_z": -42.96875,
+ "stations": "Wilhelm Mines"
+ },
+ {
+ "name": "Byllichua",
+ "pos_x": 44.9375,
+ "pos_y": -166.65625,
+ "pos_z": 155.46875,
+ "stations": "Purbach Gateway,Wolf Orbital,Lee Terminal,Cartan Base,Cardano's Inheritance,Nikitin Installation"
+ },
+ {
+ "name": "Byllit",
+ "pos_x": -112.34375,
+ "pos_y": -79.84375,
+ "pos_z": -22,
+ "stations": "Apgar Stop,Moisuc Enterprise"
+ },
+ {
+ "name": "BZ Ceti",
+ "pos_x": -10.34375,
+ "pos_y": -53.9375,
+ "pos_z": -57.59375,
+ "stations": "Coleman Ring,Finch Installation,Tull Bastion"
+ },
+ {
+ "name": "C Hydrae",
+ "pos_x": 86.125,
+ "pos_y": 40.5,
+ "pos_z": -76.84375,
+ "stations": "Butler Camp,Ogden Enterprise,Slonczewski Relay"
+ },
+ {
+ "name": "C'hi Yu",
+ "pos_x": -152.75,
+ "pos_y": -20.375,
+ "pos_z": 90.96875,
+ "stations": "Baird Settlement,Slonczewski Platform"
+ },
+ {
+ "name": "Cab",
+ "pos_x": 99.65625,
+ "pos_y": -216.03125,
+ "pos_z": -38,
+ "stations": "Elbakyan Enterprise"
+ },
+ {
+ "name": "Cabal",
+ "pos_x": -76.1875,
+ "pos_y": 96.875,
+ "pos_z": -117.71875,
+ "stations": "Boodt Platform,Xing Platform"
+ },
+ {
+ "name": "Cabalumana",
+ "pos_x": 96.6875,
+ "pos_y": 98,
+ "pos_z": -92.75,
+ "stations": "Mawson Landing,Watts Colony,Eckford Point"
+ },
+ {
+ "name": "Cabarci",
+ "pos_x": -77.65625,
+ "pos_y": -122.40625,
+ "pos_z": -103.3125,
+ "stations": "Cousin Point,Wyndham Mine,Ingstad Mines"
+ },
+ {
+ "name": "Cabassones",
+ "pos_x": -11.34375,
+ "pos_y": -97.0625,
+ "pos_z": 23.46875,
+ "stations": "Vela City"
+ },
+ {
+ "name": "Caberani",
+ "pos_x": -44.5625,
+ "pos_y": -88.0625,
+ "pos_z": -86.46875,
+ "stations": "Sheffield Dock"
+ },
+ {
+ "name": "Cabrala",
+ "pos_x": 71.59375,
+ "pos_y": -123.59375,
+ "pos_z": 147.6875,
+ "stations": "Kaneda Orbital,Lee Terminal,Gwynn Point"
+ },
+ {
+ "name": "Cabrigenses",
+ "pos_x": 77.71875,
+ "pos_y": -56.84375,
+ "pos_z": -158.46875,
+ "stations": "Wollheim Port"
+ },
+ {
+ "name": "Cabru",
+ "pos_x": 15.78125,
+ "pos_y": 80.46875,
+ "pos_z": 38,
+ "stations": "Pook City,Rice Dock,Frechet Palace,Lanier Terminal,Anvil Plant"
+ },
+ {
+ "name": "Cabrua",
+ "pos_x": 25,
+ "pos_y": -216.59375,
+ "pos_z": 34.46875,
+ "stations": "Pajdusakova Beacon"
+ },
+ {
+ "name": "Cacoma",
+ "pos_x": 110.8125,
+ "pos_y": -141.59375,
+ "pos_z": -38.78125,
+ "stations": "Hickam Orbital,Salpeter Platform,Vardeman Landing"
+ },
+ {
+ "name": "Cadu Kunus",
+ "pos_x": -128.59375,
+ "pos_y": 128.21875,
+ "pos_z": 7.09375,
+ "stations": "Larson Asylum"
+ },
+ {
+ "name": "Cadubi",
+ "pos_x": 72.46875,
+ "pos_y": -78.53125,
+ "pos_z": -59.625,
+ "stations": "Kubokawa Terminal,Bruce Penal colony,Leydenfrost Hub,Denning Vision,Gresh Installation"
+ },
+ {
+ "name": "Cadubii",
+ "pos_x": 85.4375,
+ "pos_y": -105.21875,
+ "pos_z": -64,
+ "stations": "Abe Gateway,Aikin Survey"
+ },
+ {
+ "name": "Cadupi",
+ "pos_x": 44.46875,
+ "pos_y": -58.53125,
+ "pos_z": 105.15625,
+ "stations": "Grunsfeld Station,Laming Terminal,Sewell Survey,Cassini's Progress,Clement Depot"
+ },
+ {
+ "name": "Cadupishala",
+ "pos_x": -41.25,
+ "pos_y": 73.03125,
+ "pos_z": -142.59375,
+ "stations": "Fu Dock,Diamond's Claim,Vance's Inheritance"
+ },
+ {
+ "name": "Cadurci",
+ "pos_x": -21.09375,
+ "pos_y": 120.875,
+ "pos_z": 83.5,
+ "stations": "Hamilton Sanctuary,Pullman Enterprise"
+ },
+ {
+ "name": "Caduvul",
+ "pos_x": 8.21875,
+ "pos_y": -103.6875,
+ "pos_z": 109.78125,
+ "stations": "Foss Station,Lyot Estate,Shosuke Survey"
+ },
+ {
+ "name": "Caduvun",
+ "pos_x": -46.9375,
+ "pos_y": 141.09375,
+ "pos_z": 37.84375,
+ "stations": "Blenkinsop Orbital"
+ },
+ {
+ "name": "Caduwar",
+ "pos_x": 139.84375,
+ "pos_y": -104.875,
+ "pos_z": 95.3125,
+ "stations": "Paola Outpost"
+ },
+ {
+ "name": "Caelinus",
+ "pos_x": 0.1875,
+ "pos_y": -18.625,
+ "pos_z": 52.0625,
+ "stations": "Tokubei Port,Moorcock Co-operative,Pohl Relay"
+ },
+ {
+ "name": "Caelottixa",
+ "pos_x": 6.75,
+ "pos_y": 2.09375,
+ "pos_z": 77.71875,
+ "stations": "Jones Dock,Bachman Platform"
+ },
+ {
+ "name": "Caer Doiri",
+ "pos_x": 75.0625,
+ "pos_y": -33.5,
+ "pos_z": 138.28125,
+ "stations": "Swainson City,Henry City"
+ },
+ {
+ "name": "Caer Itar",
+ "pos_x": 113.5,
+ "pos_y": -153.4375,
+ "pos_z": -23.96875,
+ "stations": "Lomonosov Prospect,Lane Survey"
+ },
+ {
+ "name": "Caeramu Tie",
+ "pos_x": -77.15625,
+ "pos_y": -49.625,
+ "pos_z": -95.53125,
+ "stations": "Broderick Settlement,Vespucci Landing,Hawkes Prospect"
+ },
+ {
+ "name": "Caerape",
+ "pos_x": 164.75,
+ "pos_y": -19.90625,
+ "pos_z": 68.9375,
+ "stations": "Vancouver Co-operative,Birmingham Dock,Lasswitz Platform,Butler's Progress"
+ },
+ {
+ "name": "Caere",
+ "pos_x": -5.84375,
+ "pos_y": -200.90625,
+ "pos_z": -13.84375,
+ "stations": "Wilson Vision,McDevitt Survey"
+ },
+ {
+ "name": "Caereda",
+ "pos_x": 106.8125,
+ "pos_y": -65.25,
+ "pos_z": 105.375,
+ "stations": "Farouk Penal colony,Elst Dock,Hirayama Installation,Dassault Gateway,Biesbroeck Hub"
+ },
+ {
+ "name": "Caereddi",
+ "pos_x": 132.875,
+ "pos_y": -9.96875,
+ "pos_z": -8.0625,
+ "stations": "Thomson Orbital,Lovell Station,Wheeler's Inheritance,Meitner's Progress,Borman Hub"
+ },
+ {
+ "name": "Caeresi",
+ "pos_x": 86.96875,
+ "pos_y": -117.65625,
+ "pos_z": 84,
+ "stations": "Gehrels Outpost"
+ },
+ {
+ "name": "Caerete",
+ "pos_x": 31.34375,
+ "pos_y": -34.375,
+ "pos_z": 135.78125,
+ "stations": "Thurston's Inheritance"
+ },
+ {
+ "name": "Caeriango",
+ "pos_x": 138.375,
+ "pos_y": -182.125,
+ "pos_z": 102.78125,
+ "stations": "Schwarzschild Hub,Stefansson Beacon,MacGill Dock,Somayaji Terminal,Russ' Claim"
+ },
+ {
+ "name": "Caeritis",
+ "pos_x": 119.8125,
+ "pos_y": -4.9375,
+ "pos_z": -23.0625,
+ "stations": "Bernoulli Vision,Avogadro Horizons,Kooi Dock"
+ },
+ {
+ "name": "Caeroerensi",
+ "pos_x": 48.84375,
+ "pos_y": 26.46875,
+ "pos_z": -139.125,
+ "stations": "Strzelecki Refinery,King Plant"
+ },
+ {
+ "name": "Caerus",
+ "pos_x": 13.125,
+ "pos_y": -27.4375,
+ "pos_z": -50.9375,
+ "stations": "Silves Terminal,Whymper City,Knight Station,Zahn Legacy,Popov Hub,Heinlein Terminal,Low's Progress,Warner Holdings,Herreshoff Arsenal"
+ },
+ {
+ "name": "Cagni",
+ "pos_x": -9.5,
+ "pos_y": -6.40625,
+ "pos_z": -168.0625,
+ "stations": "Roberts Orbital"
+ },
+ {
+ "name": "Cagnir",
+ "pos_x": 21.21875,
+ "pos_y": 143.0625,
+ "pos_z": -72.46875,
+ "stations": "Napier's Claim"
+ },
+ {
+ "name": "Cahuile",
+ "pos_x": 15.65625,
+ "pos_y": -21.5,
+ "pos_z": -60.875,
+ "stations": "Judson Installation"
+ },
+ {
+ "name": "Cai",
+ "pos_x": 89.46875,
+ "pos_y": -2.375,
+ "pos_z": -19.125,
+ "stations": "Raven's Landing,Future Hopes,Burroughs Palace,Onizuka Bastion"
+ },
+ {
+ "name": "Cai Quapa",
+ "pos_x": -103.59375,
+ "pos_y": -20.3125,
+ "pos_z": 115.15625,
+ "stations": "Markov Landing,Fowler Settlement"
+ },
+ {
+ "name": "Cai Shalang",
+ "pos_x": 52.84375,
+ "pos_y": -8.78125,
+ "pos_z": -121.15625,
+ "stations": "Lazutkin Ring"
+ },
+ {
+ "name": "Cai Syraze",
+ "pos_x": 127.40625,
+ "pos_y": -19.96875,
+ "pos_z": 65.8125,
+ "stations": "Drake Hub,Lander Penal colony,Secchi Orbital,Marcy Mines"
+ },
+ {
+ "name": "Caicius",
+ "pos_x": 41.0625,
+ "pos_y": 48.03125,
+ "pos_z": 18.96875,
+ "stations": "Heisenberg Gateway"
+ },
+ {
+ "name": "Caihe",
+ "pos_x": -58.6875,
+ "pos_y": 23.75,
+ "pos_z": 63.59375,
+ "stations": "Rennie Hub"
+ },
+ {
+ "name": "Cail",
+ "pos_x": -68.5,
+ "pos_y": -3.40625,
+ "pos_z": -140.625,
+ "stations": "Bridger Enterprise,Christian Terminal,Drummond Port,Citi's Inheritance"
+ },
+ {
+ "name": "Caill",
+ "pos_x": 85.65625,
+ "pos_y": 40.875,
+ "pos_z": 7.40625,
+ "stations": "Fleming City"
+ },
+ {
+ "name": "Caill Reddi",
+ "pos_x": -61.375,
+ "pos_y": -21.90625,
+ "pos_z": -78.25,
+ "stations": "Armstrong Station,Binnie Mines,Davy Port,Napier Works,Beaumont Market"
+ },
+ {
+ "name": "Cailla",
+ "pos_x": 25.875,
+ "pos_y": 1.875,
+ "pos_z": -107.625,
+ "stations": "Sadi Carnot Station,Simonyi City,vo Station,Haarsma Point"
+ },
+ {
+ "name": "Caillapit",
+ "pos_x": 84.15625,
+ "pos_y": 40.46875,
+ "pos_z": 103.375,
+ "stations": "Rennie Depot,Kandel Terminal,Walker Terminal"
+ },
+ {
+ "name": "Cailli",
+ "pos_x": 58.5,
+ "pos_y": -108,
+ "pos_z": 127.40625,
+ "stations": "Dillon Terminal,Delany's Inheritance,Extra Dock"
+ },
+ {
+ "name": "Caillke",
+ "pos_x": -149.34375,
+ "pos_y": 61.125,
+ "pos_z": 69.25,
+ "stations": "Hudson Retreat,Starzl's Pride"
+ },
+ {
+ "name": "Cairne",
+ "pos_x": 12.21875,
+ "pos_y": -32.5625,
+ "pos_z": -21.28125,
+ "stations": "Czerny Station,Bohr Hub"
+ },
+ {
+ "name": "Cakiniez",
+ "pos_x": 41.4375,
+ "pos_y": 82.21875,
+ "pos_z": 49.21875,
+ "stations": "Peral Lab,Vasilyev Vision"
+ },
+ {
+ "name": "Cakutsi",
+ "pos_x": -162.96875,
+ "pos_y": 26.6875,
+ "pos_z": 86.15625,
+ "stations": "Wait Colburn Dock,Vance Platform,Alexandrov Survey,Heaviside Beacon,Minkowski's Inheritance"
+ },
+ {
+ "name": "Calamari",
+ "pos_x": -18.375,
+ "pos_y": 61.5,
+ "pos_z": 100.96875,
+ "stations": "Pirsan Dock,Harvey Installation"
+ },
+ {
+ "name": "Calanggubal",
+ "pos_x": 79.1875,
+ "pos_y": -159.65625,
+ "pos_z": -63.1875,
+ "stations": "Kemurdzhian Terminal,Lambert Dock"
+ },
+ {
+ "name": "Calarum",
+ "pos_x": -62.28125,
+ "pos_y": -16.78125,
+ "pos_z": -33.09375,
+ "stations": "Darkport,Lee Terminal,Wait Colburn Hub"
+ },
+ {
+ "name": "Calch",
+ "pos_x": 111.625,
+ "pos_y": 49.75,
+ "pos_z": -56.03125,
+ "stations": "McCandless Gateway,Malpighi Port,Carter Terminal,Malaspina Survey,Goeppert-Mayer Base"
+ },
+ {
+ "name": "Calchaqui",
+ "pos_x": 38.25,
+ "pos_y": 21.5,
+ "pos_z": -97.09375,
+ "stations": "Underwood Camp,Deluc Plant"
+ },
+ {
+ "name": "Calea",
+ "pos_x": 78.78125,
+ "pos_y": -81.84375,
+ "pos_z": 45.1875,
+ "stations": "Lukyanenko Vision,Krenkel Terminal,Mechain Prospect,Baracchi City"
+ },
+ {
+ "name": "Caledo",
+ "pos_x": 99.0625,
+ "pos_y": -87.375,
+ "pos_z": 76.96875,
+ "stations": "Brosnatch Station,Chawla Terminal,Wilhelm von Struve Port,Smirnova Station,Curtiss Port,Smith Palace,Alden's Inheritance,Ziegel Vision,Westphal Station,Alexander Works,Otomo Orbital,Hewish Arsenal,Bowell Dock,Jael Port"
+ },
+ {
+ "name": "Caleket",
+ "pos_x": 31.15625,
+ "pos_y": -174.59375,
+ "pos_z": -54.21875,
+ "stations": "Cowling Refinery,Bok Base"
+ },
+ {
+ "name": "Calennero",
+ "pos_x": 104.15625,
+ "pos_y": -99.5625,
+ "pos_z": -6.15625,
+ "stations": "Bolkow Terminal"
+ },
+ {
+ "name": "Calenta",
+ "pos_x": 79.96875,
+ "pos_y": -111.75,
+ "pos_z": 78.65625,
+ "stations": "Very Hub,Bullialdus Enterprise,Dominique Relay"
+ },
+ {
+ "name": "Caleta",
+ "pos_x": -10.21875,
+ "pos_y": -86.0625,
+ "pos_z": -84,
+ "stations": "Savinykh Dock,Watson Station"
+ },
+ {
+ "name": "Calhuacan",
+ "pos_x": 69.65625,
+ "pos_y": -79.34375,
+ "pos_z": 3.125,
+ "stations": "Dearden-Salter One,Angel Station,Sakers Holdings,Bryant Bastion"
+ },
+ {
+ "name": "California Sector BA-A e6",
+ "pos_x": -319.8125,
+ "pos_y": -216.75,
+ "pos_z": -913.46875,
+ "stations": "Mic Turner Base"
+ },
+ {
+ "name": "California Sector BV-Y c7",
+ "pos_x": -342.3125,
+ "pos_y": -219.28125,
+ "pos_z": -952.71875,
+ "stations": "Darwin Research Facility"
+ },
+ {
+ "name": "California Sector CQ-Y c5",
+ "pos_x": -350.375,
+ "pos_y": -245.53125,
+ "pos_z": -950.625,
+ "stations": "The Quarry"
+ },
+ {
+ "name": "California Sector HR-W d1-28",
+ "pos_x": -329.53125,
+ "pos_y": -218.5,
+ "pos_z": -893.71875,
+ "stations": "Ford Research Laboratory"
+ },
+ {
+ "name": "California Sector JH-V c2-12",
+ "pos_x": -314.34375,
+ "pos_y": -221.71875,
+ "pos_z": -890.34375,
+ "stations": "Redmarch Laboratory"
+ },
+ {
+ "name": "Calili",
+ "pos_x": -9.78125,
+ "pos_y": 103.0625,
+ "pos_z": -47.40625,
+ "stations": "Mitchell Port,Tarski Survey"
+ },
+ {
+ "name": "Callae",
+ "pos_x": 3.3125,
+ "pos_y": -5.9375,
+ "pos_z": 128.59375,
+ "stations": "Haberlandt Point,Polyakov Base"
+ },
+ {
+ "name": "Callavs",
+ "pos_x": -16.09375,
+ "pos_y": -105.5,
+ "pos_z": -11.03125,
+ "stations": "Lindsey Installation,Kirchoff Dock"
+ },
+ {
+ "name": "Caller",
+ "pos_x": 43.875,
+ "pos_y": -10.59375,
+ "pos_z": -72.40625,
+ "stations": "Lucretius Plant,Haipeng Enterprise"
+ },
+ {
+ "name": "Callerni",
+ "pos_x": 95.40625,
+ "pos_y": -164.59375,
+ "pos_z": 126.375,
+ "stations": "Utkin Terminal"
+ },
+ {
+ "name": "Callici",
+ "pos_x": 74.875,
+ "pos_y": -20.28125,
+ "pos_z": 81.34375,
+ "stations": "Czerneda Enterprise,Eschbach Gateway,Forstchen Point"
+ },
+ {
+ "name": "Callionger",
+ "pos_x": -114.84375,
+ "pos_y": -56.46875,
+ "pos_z": -103.59375,
+ "stations": "Katzenstein Settlement,Jakes Terminal"
+ },
+ {
+ "name": "Callites",
+ "pos_x": 112.9375,
+ "pos_y": -54.4375,
+ "pos_z": 95.5,
+ "stations": "Hadid Dock,Marcy Terminal,Cardano Penal colony"
+ },
+ {
+ "name": "Callung",
+ "pos_x": 93.625,
+ "pos_y": -64.25,
+ "pos_z": -67.4375,
+ "stations": "Schomburg Beacon,Bachman Survey"
+ },
+ {
+ "name": "Calor",
+ "pos_x": -55.84375,
+ "pos_y": 7.53125,
+ "pos_z": 54.03125,
+ "stations": "Julian Hub,Khrunov Enterprise,Cleave Refinery,Papin Terminal,Ziemianski Penal colony"
+ },
+ {
+ "name": "Calou",
+ "pos_x": 157,
+ "pos_y": -76.1875,
+ "pos_z": 64.5,
+ "stations": "Fukushima Vision,Hottot Reach,Bharadwaj Landing"
+ },
+ {
+ "name": "Calourotep",
+ "pos_x": 123.65625,
+ "pos_y": -74.65625,
+ "pos_z": 73.625,
+ "stations": "Adelman Colony,Mechain Depot"
+ },
+ {
+ "name": "Calugen",
+ "pos_x": -106.03125,
+ "pos_y": 65,
+ "pos_z": 27.46875,
+ "stations": "Bloomfield Gateway,Edgeworth Station,Lasswitz Installation,Arkwright Station"
+ },
+ {
+ "name": "Cama",
+ "pos_x": -47.0625,
+ "pos_y": 42.84375,
+ "pos_z": 30.5,
+ "stations": "Korzun Station,Rae Lab,Gauss Hub,Jacquard Gateway,Yeliseyev City,Selye Dock,Eddington Market,Carson Depot"
+ },
+ {
+ "name": "Cama Zotz",
+ "pos_x": 42.71875,
+ "pos_y": -47.125,
+ "pos_z": -45.5,
+ "stations": "Drake Penal colony,Stanier Gateway,Braun Installation,Robson Survey,Anders Dock"
+ },
+ {
+ "name": "Camab",
+ "pos_x": 76.25,
+ "pos_y": 97.28125,
+ "pos_z": 100.03125,
+ "stations": "Macan Port,Lasswitz Vista"
+ },
+ {
+ "name": "Camaitsogo",
+ "pos_x": -51.34375,
+ "pos_y": 105.46875,
+ "pos_z": 23,
+ "stations": "Crown Enterprise,Kuttner Terminal,Brackett Ring,Coney Keep,Fabian Laboratory"
+ },
+ {
+ "name": "Camarica",
+ "pos_x": 5.9375,
+ "pos_y": 1.78125,
+ "pos_z": -142.9375,
+ "stations": "Leibniz Dock,Fowler Base,Voss Terminal,McQuay's Claim"
+ },
+ {
+ "name": "Camat",
+ "pos_x": 103.90625,
+ "pos_y": -164.09375,
+ "pos_z": 2.9375,
+ "stations": "Araki Port,Bigourdan Terminal,Johnson Orbital,Wood Survey"
+ },
+ {
+ "name": "Camaye",
+ "pos_x": -105.46875,
+ "pos_y": 41.5625,
+ "pos_z": -104.71875,
+ "stations": "Molina Orbital,Chaviano Works,Winne Station,Szebehely Station"
+ },
+ {
+ "name": "Camazotz",
+ "pos_x": 38.15625,
+ "pos_y": 12.65625,
+ "pos_z": 97.375,
+ "stations": "Swanwick Station,Forstchen Dock,Ballard Hub,Chilton Enterprise,Nicholson Observatory,Champlain Terminal,McAllaster Hub,Lagrange Station,Griffith Dock,Brust Camp,Stross Depot,Roberts Relay,Santos Installation,Jeschke Oasis"
+ },
+ {
+ "name": "Cambal",
+ "pos_x": 16.8125,
+ "pos_y": 144.09375,
+ "pos_z": -4.96875,
+ "stations": "Bethke Hub,Derleth Horizons,Dalton Penal colony"
+ },
+ {
+ "name": "Cambo",
+ "pos_x": 101.875,
+ "pos_y": -130.125,
+ "pos_z": 143.28125,
+ "stations": "Arend City"
+ },
+ {
+ "name": "Cambou",
+ "pos_x": -91.5625,
+ "pos_y": 60.5,
+ "pos_z": 124.0625,
+ "stations": "Musabayev Hangar,Wyeth Settlement"
+ },
+ {
+ "name": "Came",
+ "pos_x": -164.28125,
+ "pos_y": 15.15625,
+ "pos_z": 68.4375,
+ "stations": "MacLean Platform,Lovell Mines,Efremov Reach"
+ },
+ {
+ "name": "Camenisar",
+ "pos_x": -157.03125,
+ "pos_y": -75.9375,
+ "pos_z": -25.5625,
+ "stations": "Williams Dock,Willis Landing"
+ },
+ {
+ "name": "Campanisar",
+ "pos_x": -154.5625,
+ "pos_y": -3.71875,
+ "pos_z": 81.46875,
+ "stations": "Brooks' Claim,Ellern Arena,Selberg Terminal"
+ },
+ {
+ "name": "Campi",
+ "pos_x": -42.4375,
+ "pos_y": 64.375,
+ "pos_z": 105.5,
+ "stations": "Eudoxus Retreat,Drake's Folly"
+ },
+ {
+ "name": "Camulus",
+ "pos_x": 3.125,
+ "pos_y": -135.5625,
+ "pos_z": -62.96875,
+ "stations": "Gaultier de Varennes Settlement,Smith Refinery,Bauschinger Settlement,Converse Refinery"
+ },
+ {
+ "name": "Camumba",
+ "pos_x": 28.875,
+ "pos_y": -37.3125,
+ "pos_z": 92.78125,
+ "stations": "Pordenone's Folly"
+ },
+ {
+ "name": "Camunab",
+ "pos_x": -15.9375,
+ "pos_y": -178.625,
+ "pos_z": 128.25,
+ "stations": "Melotte Base,Newcomb Prospect"
+ },
+ {
+ "name": "Camundjiga",
+ "pos_x": 76.3125,
+ "pos_y": -190.28125,
+ "pos_z": 101.15625,
+ "stations": "Wheeler Vision,Dubyago Landing,Bendell Terminal"
+ },
+ {
+ "name": "Camundju",
+ "pos_x": 157.75,
+ "pos_y": -136.0625,
+ "pos_z": 100.59375,
+ "stations": "Vasquez de Coronado Base,Evans Enterprise,Foster Terminal"
+ },
+ {
+ "name": "Camuten",
+ "pos_x": 158.40625,
+ "pos_y": -67.375,
+ "pos_z": 47.28125,
+ "stations": "Riess Colony,Tisserand Hub,Wallin Beacon,Apianus Dock,Baydukov Silo"
+ },
+ {
+ "name": "Can Hut",
+ "pos_x": 83.34375,
+ "pos_y": 67.46875,
+ "pos_z": -126.21875,
+ "stations": "al-Haytham Outpost"
+ },
+ {
+ "name": "Can Nik",
+ "pos_x": -153.90625,
+ "pos_y": 32.46875,
+ "pos_z": -53.71875,
+ "stations": "Slusser Camp"
+ },
+ {
+ "name": "Can Qing",
+ "pos_x": 59.71875,
+ "pos_y": -185.8125,
+ "pos_z": 102.6875,
+ "stations": "William Sargent Hub,Moffat Hub,Marques Survey,Reeves Platform"
+ },
+ {
+ "name": "Can Zu",
+ "pos_x": -40.53125,
+ "pos_y": -11.375,
+ "pos_z": 141.1875,
+ "stations": "Dann's Claim"
+ },
+ {
+ "name": "Canaharvas",
+ "pos_x": 138.46875,
+ "pos_y": 21.6875,
+ "pos_z": -91.40625,
+ "stations": "Tilley Retreat"
+ },
+ {
+ "name": "Canahati",
+ "pos_x": 100.28125,
+ "pos_y": -131.09375,
+ "pos_z": -55.0625,
+ "stations": "Witt's Folly,Jones Port,Lintott Vision"
+ },
+ {
+ "name": "Canakuts",
+ "pos_x": -14.125,
+ "pos_y": -174.875,
+ "pos_z": 88.09375,
+ "stations": "Thomson Beacon,Spurzem Works"
+ },
+ {
+ "name": "Canani",
+ "pos_x": 52.59375,
+ "pos_y": -125.6875,
+ "pos_z": 51.46875,
+ "stations": "Nomura Orbital"
+ },
+ {
+ "name": "Canari",
+ "pos_x": 99.28125,
+ "pos_y": -41.8125,
+ "pos_z": -75.53125,
+ "stations": "O'Connor Orbital,Pawelczyk Terminal,Nyberg Dock,Humphreys Point"
+ },
+ {
+ "name": "Canarigui",
+ "pos_x": -21.15625,
+ "pos_y": -105.125,
+ "pos_z": 11.46875,
+ "stations": "Mayer Vision,Flamsteed Horizons,Alpers Arsenal,Pelt Relay"
+ },
+ {
+ "name": "Canda",
+ "pos_x": 56.375,
+ "pos_y": -198.6875,
+ "pos_z": 66.09375,
+ "stations": "Akiyama Mines,Seyfert Survey"
+ },
+ {
+ "name": "Candecama",
+ "pos_x": 56.75,
+ "pos_y": -99.1875,
+ "pos_z": 84.34375,
+ "stations": "Oshima Dock,Whelan Ring,Sheremetevsky City,Pennington Hub,Otus Orbital,Horrocks Gateway,Vogel Hub,Puleston Relay,Burns Hub,Herjulfsson's Claim,Svavarsson Vision,Thornycroft Landing,Whitford Relay,Rosse Orbital,Mattei Exchange"
+ },
+ {
+ "name": "Candhari",
+ "pos_x": 12.71875,
+ "pos_y": -118.09375,
+ "pos_z": -173.0625,
+ "stations": "Kolmogorov Freeport,Larson Survey,Yolen Base"
+ },
+ {
+ "name": "Candi",
+ "pos_x": 101.9375,
+ "pos_y": -74.6875,
+ "pos_z": -82.5625,
+ "stations": "Ramaswamy Mines"
+ },
+ {
+ "name": "Candiae",
+ "pos_x": 31.84375,
+ "pos_y": -187.375,
+ "pos_z": 3.09375,
+ "stations": "Baille Survey,Wachmann Colony"
+ },
+ {
+ "name": "Candiaei",
+ "pos_x": -113.5,
+ "pos_y": -4.9375,
+ "pos_z": 66.84375,
+ "stations": "Crown Terminal,Lloyd Dock,Lem Dock,Ledyard Hub,RenenBellot Port,Powers City,Donaldson Terminal,Narita Hub,Wilson Dock,Pauling Landing,Hume Prospect,Niven Vision,Barcelo Prospect"
+ },
+ {
+ "name": "Candin",
+ "pos_x": 103.875,
+ "pos_y": 129.625,
+ "pos_z": 26.65625,
+ "stations": "Galvani Enterprise,Gorbatko Port"
+ },
+ {
+ "name": "Candjinan",
+ "pos_x": -143.25,
+ "pos_y": 30.9375,
+ "pos_z": -68.6875,
+ "stations": "Pippin Port,Temple Works,Harrison Terminal"
+ },
+ {
+ "name": "Candra",
+ "pos_x": -119.65625,
+ "pos_y": -72.1875,
+ "pos_z": 57,
+ "stations": "Wohler Horizons"
+ },
+ {
+ "name": "Caneph",
+ "pos_x": 93.65625,
+ "pos_y": -124.625,
+ "pos_z": 142.15625,
+ "stations": "Lippisch Point,Kludze Beacon"
+ },
+ {
+ "name": "Canezti",
+ "pos_x": -60.84375,
+ "pos_y": -4.9375,
+ "pos_z": 96.4375,
+ "stations": "Stewart Port,Murray Vision,Shriver City,Tenn Penal colony"
+ },
+ {
+ "name": "Canis Subridens",
+ "pos_x": -9502.125,
+ "pos_y": -921.3125,
+ "pos_z": 19784.96875,
+ "stations": "The Bone Yard"
+ },
+ {
+ "name": "Canonnia",
+ "pos_x": -9522.9375,
+ "pos_y": -894.0625,
+ "pos_z": 19791.875,
+ "stations": "Arcanonn's Legacy"
+ },
+ {
+ "name": "Canopus",
+ "pos_x": 276.625,
+ "pos_y": -131.34375,
+ "pos_z": -42.4375,
+ "stations": "Loncke Keep,Rangarajan's Base"
+ },
+ {
+ "name": "Cant",
+ "pos_x": 126.40625,
+ "pos_y": -249.03125,
+ "pos_z": 87.78125,
+ "stations": "Schaumasse Prospect"
+ },
+ {
+ "name": "Canta Athis",
+ "pos_x": 49.28125,
+ "pos_y": -102,
+ "pos_z": 107.71875,
+ "stations": "Smirnova Enterprise,Carnera Station,Fokker Ring,Bertin Relay"
+ },
+ {
+ "name": "Cantei",
+ "pos_x": 107.59375,
+ "pos_y": -217.09375,
+ "pos_z": 95.75,
+ "stations": "Vakhmistrov Legacy,Roche Installation"
+ },
+ {
+ "name": "Cantiaci",
+ "pos_x": 35.1875,
+ "pos_y": -178.875,
+ "pos_z": -77.5625,
+ "stations": "Fabricius Colony,Jung Bastion,Dhawan Vision"
+ },
+ {
+ "name": "Cantine",
+ "pos_x": 127.78125,
+ "pos_y": -129.875,
+ "pos_z": 100.125,
+ "stations": "Friedrich Peters Depot,Peebles Relay"
+ },
+ {
+ "name": "Cantjarisni",
+ "pos_x": -45.09375,
+ "pos_y": 43.5625,
+ "pos_z": 82.6875,
+ "stations": "Cochrane Enterprise,Kimberlin Ring,Tepper City"
+ },
+ {
+ "name": "Canto",
+ "pos_x": 138.375,
+ "pos_y": -89.34375,
+ "pos_z": 99,
+ "stations": "May's Claim"
+ },
+ {
+ "name": "Cantsu",
+ "pos_x": 154,
+ "pos_y": 3.59375,
+ "pos_z": -19.625,
+ "stations": "Grigson Survey,Manarov Relay"
+ },
+ {
+ "name": "Canty",
+ "pos_x": 46.59375,
+ "pos_y": 14.71875,
+ "pos_z": 153.0625,
+ "stations": "Lee Platform,Emshwiller Platform"
+ },
+ {
+ "name": "Cantzicnal",
+ "pos_x": 192.875,
+ "pos_y": -177.6875,
+ "pos_z": 8.5,
+ "stations": "Islam Orbital,Covington Port,Scotti Landing"
+ },
+ {
+ "name": "Cao Gong",
+ "pos_x": -14.59375,
+ "pos_y": -222.78125,
+ "pos_z": -15.3125,
+ "stations": "Qushji Terminal,Vess Ring,Yuzhe Depot"
+ },
+ {
+ "name": "Cao Guatun",
+ "pos_x": 82.90625,
+ "pos_y": -3.1875,
+ "pos_z": 80.4375,
+ "stations": "Ross Port,Blaylock Gateway,Barnard Holdings,Aitken Barracks,Speke Port"
+ },
+ {
+ "name": "Cao Guojiu",
+ "pos_x": -62.0625,
+ "pos_y": 61.21875,
+ "pos_z": 55.96875,
+ "stations": "Poleshchuk Port,Grunsfeld Platform"
+ },
+ {
+ "name": "Cao Junga",
+ "pos_x": -139.4375,
+ "pos_y": 7.46875,
+ "pos_z": -108.53125,
+ "stations": "Szilard Point,Soddy Port"
+ },
+ {
+ "name": "Cao Tzu",
+ "pos_x": 71.6875,
+ "pos_y": -74.46875,
+ "pos_z": -135.5,
+ "stations": "Tsibliyev Settlement,Lopez-Alegria Dock,Borlaug Installation"
+ },
+ {
+ "name": "Cao Yankir",
+ "pos_x": -57.90625,
+ "pos_y": -101.90625,
+ "pos_z": -9.75,
+ "stations": "Good Gateway"
+ },
+ {
+ "name": "Cao Yans",
+ "pos_x": -74.03125,
+ "pos_y": 57,
+ "pos_z": 14.71875,
+ "stations": "Nordenskiold Hub"
+ },
+ {
+ "name": "Capa",
+ "pos_x": 60.75,
+ "pos_y": 48.65625,
+ "pos_z": -174.53125,
+ "stations": "Godwin Dock"
+ },
+ {
+ "name": "Capai",
+ "pos_x": 26.125,
+ "pos_y": -161.09375,
+ "pos_z": -80.125,
+ "stations": "Wheeler Prospect,Extra Landing,Dunyach Horizons,Tietjen Laboratory"
+ },
+ {
+ "name": "Capella",
+ "pos_x": -12.53125,
+ "pos_y": 3.8125,
+ "pos_z": -40.78125,
+ "stations": "Darkesport,Schilling Market,Schmidt Orbiter,Matthews Base,Asimov Vista,Lowry Market,Charles Orbiter,Dedman Holdings"
+ },
+ {
+ "name": "Caph",
+ "pos_x": -48.3125,
+ "pos_y": -2.9375,
+ "pos_z": -25.59375,
+ "stations": "Maclaurin Terminal,Friend Observatory,Wells Barracks"
+ },
+ {
+ "name": "Capo",
+ "pos_x": 41.59375,
+ "pos_y": -39.96875,
+ "pos_z": -71.9375,
+ "stations": "Grothendieck Penal colony,Britnev Terminal,McCaffrey City,Ray Vision"
+ },
+ {
+ "name": "Capoos",
+ "pos_x": 51.71875,
+ "pos_y": -49.71875,
+ "pos_z": -80.71875,
+ "stations": "Avogadro Ring,Hirase Enterprise,Winne Laboratory"
+ },
+ {
+ "name": "Capoya",
+ "pos_x": -60.65625,
+ "pos_y": 82.4375,
+ "pos_z": -45.0625,
+ "stations": "Brin Port,Wilhelm Station,Scott's Pride,Kennan Exchange"
+ },
+ {
+ "name": "Capricorni Sector BL-X b1-3",
+ "pos_x": -59.375,
+ "pos_y": -73.9375,
+ "pos_z": 87.34375,
+ "stations": "Gann Base,Vonarburg Base"
+ },
+ {
+ "name": "Capricorni Sector CG-X b1-5",
+ "pos_x": -76.21875,
+ "pos_y": -104.90625,
+ "pos_z": 79.28125,
+ "stations": "Piccard Plant,Boulle Enterprise"
+ },
+ {
+ "name": "Capricorni Sector CL-Y c12",
+ "pos_x": -115.15625,
+ "pos_y": -116.375,
+ "pos_z": 89.8125,
+ "stations": "Clark's Folly"
+ },
+ {
+ "name": "Capricorni Sector CL-Y d113",
+ "pos_x": -86.6875,
+ "pos_y": -87.9375,
+ "pos_z": 72.59375,
+ "stations": "Moore Landing,Wul Laboratory"
+ },
+ {
+ "name": "Capricorni Sector DB-X b1-3",
+ "pos_x": -103.28125,
+ "pos_y": -111.5625,
+ "pos_z": 86.78125,
+ "stations": "Drew Colony"
+ },
+ {
+ "name": "Capricorni Sector DR-V b2-0",
+ "pos_x": -102.5,
+ "pos_y": -81.28125,
+ "pos_z": 98.40625,
+ "stations": "Wilder Terminal"
+ },
+ {
+ "name": "Capricorni Sector EG-Y d108",
+ "pos_x": -85.875,
+ "pos_y": -123.21875,
+ "pos_z": 111.75,
+ "stations": "Brule Base,Lee Stop"
+ },
+ {
+ "name": "Capricorni Sector EG-Y d72",
+ "pos_x": -95.625,
+ "pos_y": -138,
+ "pos_z": 104.3125,
+ "stations": "Elcano Landing"
+ },
+ {
+ "name": "Capricorni Sector FM-V b2-0",
+ "pos_x": -103.59375,
+ "pos_y": -100.375,
+ "pos_z": 97.4375,
+ "stations": "The Armoured Saint"
+ },
+ {
+ "name": "Capricorni Sector FW-W c1-11",
+ "pos_x": -73.1875,
+ "pos_y": -92.3125,
+ "pos_z": 108.15625,
+ "stations": "MacLeod Base"
+ },
+ {
+ "name": "Capricorni Sector FW-W c1-15",
+ "pos_x": -81.71875,
+ "pos_y": -78.0625,
+ "pos_z": 115.3125,
+ "stations": "Doctorow Relay,Platt Landing"
+ },
+ {
+ "name": "Capricorni Sector FW-W c1-21",
+ "pos_x": -69.65625,
+ "pos_y": -71.4375,
+ "pos_z": 125.5,
+ "stations": "Steinmuller's Progress,Wiener Installation"
+ },
+ {
+ "name": "Capricorni Sector FW-W c1-27",
+ "pos_x": -86.09375,
+ "pos_y": -99.21875,
+ "pos_z": 124.375,
+ "stations": "Clough Prospect"
+ },
+ {
+ "name": "Capricorni Sector HR-W c1-19",
+ "pos_x": -77.5,
+ "pos_y": -137.4375,
+ "pos_z": 103.1875,
+ "stations": "Trinh Base,Shatner Lab"
+ },
+ {
+ "name": "Capricorni Sector HR-W c1-22",
+ "pos_x": -91.375,
+ "pos_y": -134.46875,
+ "pos_z": 105.78125,
+ "stations": "Macgregor Lab"
+ },
+ {
+ "name": "Capricorni Sector HR-W c1-9",
+ "pos_x": -65.96875,
+ "pos_y": -140.8125,
+ "pos_z": 117.9375,
+ "stations": "Nagata Plant,Plexico Vision"
+ },
+ {
+ "name": "Capricorni Sector IH-V b2-1",
+ "pos_x": -78.78125,
+ "pos_y": -123.46875,
+ "pos_z": 104.625,
+ "stations": "Corte-Real Base"
+ },
+ {
+ "name": "Capricorni Sector JC-V c2-3",
+ "pos_x": -73.21875,
+ "pos_y": -102,
+ "pos_z": 149.84375,
+ "stations": "Poisson's Pride"
+ },
+ {
+ "name": "Capricorni Sector KC-V c2-29",
+ "pos_x": -37.59375,
+ "pos_y": -99.8125,
+ "pos_z": 136.78125,
+ "stations": "Tavares Escape,Tudela Oasis"
+ },
+ {
+ "name": "Capricorni Sector KC-V c2-31",
+ "pos_x": -41.84375,
+ "pos_y": -89.34375,
+ "pos_z": 144.34375,
+ "stations": "Everest Oasis"
+ },
+ {
+ "name": "Capricorni Sector NN-T b3-1",
+ "pos_x": -55.3125,
+ "pos_y": -116.15625,
+ "pos_z": 124.84375,
+ "stations": "Morgan Prospect"
+ },
+ {
+ "name": "Capukanga",
+ "pos_x": -26.09375,
+ "pos_y": -22.25,
+ "pos_z": -63.28125,
+ "stations": "Melnick Station,Brooks Arsenal,Scheutz Terminal,Bentham Horizons"
+ },
+ {
+ "name": "Capura",
+ "pos_x": -143.375,
+ "pos_y": 62.375,
+ "pos_z": -3.21875,
+ "stations": "Lamarck Settlement,Thuot Settlement"
+ },
+ {
+ "name": "Cara",
+ "pos_x": 72.5,
+ "pos_y": 106.78125,
+ "pos_z": -108.75,
+ "stations": "Kapp Mine"
+ },
+ {
+ "name": "Caraceni",
+ "pos_x": -49.40625,
+ "pos_y": 63.6875,
+ "pos_z": -49.3125,
+ "stations": "Kerr Enterprise,Fancher Terminal,Fairbairn Gateway,Carleson Installation,Weyl's Progress"
+ },
+ {
+ "name": "Carang Hut",
+ "pos_x": 17.0625,
+ "pos_y": 118.875,
+ "pos_z": 15.71875,
+ "stations": "Hassanein Legacy,Quimper Vision"
+ },
+ {
+ "name": "Caraswang",
+ "pos_x": 77.46875,
+ "pos_y": 1.78125,
+ "pos_z": -3.9375,
+ "stations": "vo Colony,Northrop Landing"
+ },
+ {
+ "name": "Carcinus",
+ "pos_x": 60.6875,
+ "pos_y": -33.84375,
+ "pos_z": 39.875,
+ "stations": "Wye-Delta Station,Solovyev Colony,Crook's Progress"
+ },
+ {
+ "name": "Carcosa",
+ "pos_x": -9544.25,
+ "pos_y": -879.59375,
+ "pos_z": 19822.03125,
+ "stations": "Robardin Rock,Aragon,Amber Dock"
+ },
+ {
+ "name": "Cardea",
+ "pos_x": 94.1875,
+ "pos_y": -106.03125,
+ "pos_z": 21.09375,
+ "stations": "Tygerlily-Merlyn Pleasuredome,Raymo's R & R & R,Utkin Palace,Narbeth Holdings"
+ },
+ {
+ "name": "Carener",
+ "pos_x": -5.5625,
+ "pos_y": -14.625,
+ "pos_z": 15.28125,
+ "stations": "Butz Port,Bates Horizons"
+ },
+ {
+ "name": "Carenes",
+ "pos_x": -83.5,
+ "pos_y": -2.9375,
+ "pos_z": -80.90625,
+ "stations": "Buckland Colony,Smith Colony,Cabana's Inheritance,Pauling Plant"
+ },
+ {
+ "name": "Cares",
+ "pos_x": 78.84375,
+ "pos_y": -62.6875,
+ "pos_z": 140.71875,
+ "stations": "Lyot Port,Pittendreigh Enterprise,Daqing Orbital,Hoshi Enterprise,Dozois Enterprise"
+ },
+ {
+ "name": "Caret Werek",
+ "pos_x": 206.125,
+ "pos_y": -178.5,
+ "pos_z": -24.96875,
+ "stations": "Montrose Horizons,Fieseler Port"
+ },
+ {
+ "name": "Cari",
+ "pos_x": 90.4375,
+ "pos_y": -84.15625,
+ "pos_z": 83.09375,
+ "stations": "Michael Station,van de Hulst Dock,Elson Vision"
+ },
+ {
+ "name": "Caria",
+ "pos_x": 59.40625,
+ "pos_y": -123.75,
+ "pos_z": -73.46875,
+ "stations": "Somerville Station"
+ },
+ {
+ "name": "Cariangan",
+ "pos_x": 1.1875,
+ "pos_y": -93.21875,
+ "pos_z": -40.15625,
+ "stations": "Hollander Prospect,Sargent Beacon,Pond Laboratory,Lozino-Lozinskiy Vision"
+ },
+ {
+ "name": "Cariaya",
+ "pos_x": -83.90625,
+ "pos_y": -33.5625,
+ "pos_z": 86.4375,
+ "stations": "Howard Depot"
+ },
+ {
+ "name": "Cariba",
+ "pos_x": 45.03125,
+ "pos_y": -162.5,
+ "pos_z": -81.5625,
+ "stations": "Bowen Base,Cook Beacon"
+ },
+ {
+ "name": "Caribri",
+ "pos_x": -23,
+ "pos_y": -46,
+ "pos_z": -11.875,
+ "stations": "Helmholtz Orbital,Coke City,Gaultier de Varennes Legacy,Joule Orbital"
+ },
+ {
+ "name": "Carichilo",
+ "pos_x": 15.875,
+ "pos_y": -32.90625,
+ "pos_z": -62.03125,
+ "stations": "Berezovoy Terminal,Clark Enterprise,Meredith's Inheritance,Potrykus Port,Simonyi Depot"
+ },
+ {
+ "name": "Carici",
+ "pos_x": -157.5,
+ "pos_y": -67.46875,
+ "pos_z": 15.5,
+ "stations": "Hopkinson Orbital,Samuda Port,Roberts Colony,Volterra Legacy,McMullen Depot,Tall's Folly"
+ },
+ {
+ "name": "Carii",
+ "pos_x": -60.53125,
+ "pos_y": 43.71875,
+ "pos_z": -123.46875,
+ "stations": "Hottot Beacon,Barbosa's Claim"
+ },
+ {
+ "name": "Carini",
+ "pos_x": 88.59375,
+ "pos_y": -3.09375,
+ "pos_z": 1.46875,
+ "stations": "Hughes City,Chalker Landing,Roberts Dock,Lawhead's Progress,Bogdanov Settlement,Malkendorf"
+ },
+ {
+ "name": "Caripurunma",
+ "pos_x": 31.84375,
+ "pos_y": -146.375,
+ "pos_z": -69.625,
+ "stations": "Balog Point"
+ },
+ {
+ "name": "Caris",
+ "pos_x": -87.5,
+ "pos_y": 2.4375,
+ "pos_z": 51.875,
+ "stations": "Lorenz Dock"
+ },
+ {
+ "name": "Caritra",
+ "pos_x": 37.75,
+ "pos_y": -1.125,
+ "pos_z": 99.0625,
+ "stations": "Cseszneky Dock"
+ },
+ {
+ "name": "Carlota",
+ "pos_x": -9531.40625,
+ "pos_y": -874.96875,
+ "pos_z": 19797.53125,
+ "stations": "Cubil del Lobo"
+ },
+ {
+ "name": "Carmenta",
+ "pos_x": -15.6875,
+ "pos_y": -40.5,
+ "pos_z": 39.375,
+ "stations": "Eyharts Terminal,Darlton Reach,Vernadsky Dock,Hartsfield Dock"
+ },
+ {
+ "name": "Carnai",
+ "pos_x": 78.34375,
+ "pos_y": -83.40625,
+ "pos_z": 100.96875,
+ "stations": "Lyot Enterprise,McIntosh Terminal,Battani Station,Goodman Enterprise"
+ },
+ {
+ "name": "Carnaring",
+ "pos_x": 35.8125,
+ "pos_y": -81.53125,
+ "pos_z": 137.34375,
+ "stations": "Herzog Base,Jones Refinery"
+ },
+ {
+ "name": "Carnates",
+ "pos_x": -47.96875,
+ "pos_y": -47.59375,
+ "pos_z": -139.59375,
+ "stations": "Houtman Station,Bradshaw Beacon,Gardner Beacon"
+ },
+ {
+ "name": "Carnatla",
+ "pos_x": 43.8125,
+ "pos_y": 165.0625,
+ "pos_z": 43.96875,
+ "stations": "Svavarsson Asylum"
+ },
+ {
+ "name": "Carnaur",
+ "pos_x": 29.6875,
+ "pos_y": -163.1875,
+ "pos_z": 100.1875,
+ "stations": "Hewish Ring,Johnson Station,Cowell Port,Johri Vision,Anastase Perrotin Dock,Mayer Dock,Drzewiecki Vision,Zoline Bastion,Giacconi Terminal"
+ },
+ {
+ "name": "Carnoeck",
+ "pos_x": 35.96875,
+ "pos_y": 5.1875,
+ "pos_z": 1.3125,
+ "stations": "Mitchell Ring,Bacon City,Midgley Terminal,Zelazny's Progress"
+ },
+ {
+ "name": "Carnonacae",
+ "pos_x": 139.03125,
+ "pos_y": -28.15625,
+ "pos_z": 46.9375,
+ "stations": "Chacornac Settlement"
+ },
+ {
+ "name": "Carns",
+ "pos_x": 146.6875,
+ "pos_y": -97.1875,
+ "pos_z": 77.25,
+ "stations": "Humason Orbital,Christy Terminal,Abasheli Legacy,Shaw Station"
+ },
+ {
+ "name": "Carnsan",
+ "pos_x": -69.4375,
+ "pos_y": 42,
+ "pos_z": -52.4375,
+ "stations": "Boas Station,Bykovsky Hub,Kuipers Orbital,Broglie Settlement"
+ },
+ {
+ "name": "Carnutet",
+ "pos_x": 121.1875,
+ "pos_y": -103.25,
+ "pos_z": -38.8125,
+ "stations": "Harvey-Smith Camp"
+ },
+ {
+ "name": "Carpaka",
+ "pos_x": 67.9375,
+ "pos_y": -64.78125,
+ "pos_z": -69.6875,
+ "stations": "Anderson's Inheritance,Whitney Dock,Romanenko Hub,Archimedes Terminal"
+ },
+ {
+ "name": "Carpako",
+ "pos_x": 144.625,
+ "pos_y": -141.25,
+ "pos_z": 94.625,
+ "stations": "Palmer Stop,Canty Mine"
+ },
+ {
+ "name": "Carpaso",
+ "pos_x": 53.0625,
+ "pos_y": 73.15625,
+ "pos_z": -72.625,
+ "stations": "Borisenko Orbital,Swainson Terminal,Gwynn Survey,Lazutkin Port"
+ },
+ {
+ "name": "Carpetani",
+ "pos_x": 37.25,
+ "pos_y": -45.15625,
+ "pos_z": -101,
+ "stations": "Maxwell Enterprise,Avdeyev Enterprise,Bernard Ring,Bretnor Terminal"
+ },
+ {
+ "name": "Carpuli",
+ "pos_x": -23.71875,
+ "pos_y": -104.34375,
+ "pos_z": -92.40625,
+ "stations": "Bethe Camp,Anders Port,Tani Hub"
+ },
+ {
+ "name": "Carpulia",
+ "pos_x": -147.28125,
+ "pos_y": 28.4375,
+ "pos_z": 36.03125,
+ "stations": "Harris Dock"
+ },
+ {
+ "name": "Carrua",
+ "pos_x": -39.15625,
+ "pos_y": -131.28125,
+ "pos_z": 97.71875,
+ "stations": "Krigstein Holdings,Bowen Dock,Chadbourne Dock,Paez Landing"
+ },
+ {
+ "name": "Cartama",
+ "pos_x": -16.78125,
+ "pos_y": -138.71875,
+ "pos_z": 57.8125,
+ "stations": "Pannekoek Platform,Hoffmeister Vision,Yano Point"
+ },
+ {
+ "name": "Cartaro",
+ "pos_x": 95.59375,
+ "pos_y": -42.53125,
+ "pos_z": 77.3125,
+ "stations": "August von Steinheil Base"
+ },
+ {
+ "name": "Carthage",
+ "pos_x": 100.34375,
+ "pos_y": -102.125,
+ "pos_z": 7.34375,
+ "stations": "Cook Depot,Marker Depot,Port Elissa,Radwar Enterprises 1941"
+ },
+ {
+ "name": "Cartoi",
+ "pos_x": 155.96875,
+ "pos_y": -110.75,
+ "pos_z": 29.71875,
+ "stations": "Alexander Terminal,Zhuravleva Dock,Giancola Terminal,Puiseux Dock,Dittmar Orbital"
+ },
+ {
+ "name": "Cartoq",
+ "pos_x": 45.625,
+ "pos_y": 39.4375,
+ "pos_z": 60.46875,
+ "stations": "Cogswell Vision,Avdeyev Settlement,Rey Hub"
+ },
+ {
+ "name": "Cartu",
+ "pos_x": 81.25,
+ "pos_y": -58.46875,
+ "pos_z": 26.96875,
+ "stations": "Hughes Gateway,Giraud Ring,Anderson Vision,Pournelle's Folly,Tiedemann Keep"
+ },
+ {
+ "name": "Caru",
+ "pos_x": 125.75,
+ "pos_y": 3.1875,
+ "pos_z": 127,
+ "stations": "Bowen Settlement,England Colony,Bunch Prospect"
+ },
+ {
+ "name": "Carughman",
+ "pos_x": 94.09375,
+ "pos_y": 81.40625,
+ "pos_z": -45.5625,
+ "stations": "McDivitt Dock"
+ },
+ {
+ "name": "Carum",
+ "pos_x": 27.46875,
+ "pos_y": 72.15625,
+ "pos_z": 89.6875,
+ "stations": "Wrangel Port,Norman Works,Clute Hub,Sinisalo Landing,Mouhot Hub"
+ },
+ {
+ "name": "Carunda",
+ "pos_x": -108.46875,
+ "pos_y": 109.53125,
+ "pos_z": -35.03125,
+ "stations": "Nicolet Colony,Sekelj Settlement,Rothfuss Dock"
+ },
+ {
+ "name": "Carverda",
+ "pos_x": 101.84375,
+ "pos_y": -130,
+ "pos_z": -40.625,
+ "stations": "Theodorsen Port,McKie City,Luyten Ring,Maire's Inheritance,Adragna Terminal,d'Arrest Vision,Kowal Base,Wilder Palace,Bouwens' Claim"
+ },
+ {
+ "name": "Carvii",
+ "pos_x": 70.34375,
+ "pos_y": -63.0625,
+ "pos_z": 122.65625,
+ "stations": "Kirshner Relay"
+ },
+ {
+ "name": "Cashibo",
+ "pos_x": -39.90625,
+ "pos_y": 43.9375,
+ "pos_z": 135.3125,
+ "stations": "Nagel Enterprise"
+ },
+ {
+ "name": "Cashikori",
+ "pos_x": 105.09375,
+ "pos_y": -16.5625,
+ "pos_z": 114.53125,
+ "stations": "Angstrom Terminal,Covington Base,Russell Mines"
+ },
+ {
+ "name": "Cashis",
+ "pos_x": 87.71875,
+ "pos_y": 29.625,
+ "pos_z": 152.59375,
+ "stations": "Grunsfeld Horizons,Hahn Dock,Al-Farabi Colony,Wrangell Keep,Beltrami Enterprise,Gessi Prospect"
+ },
+ {
+ "name": "Casmatyas",
+ "pos_x": 69.78125,
+ "pos_y": -56.8125,
+ "pos_z": 101.5625,
+ "stations": "Muramatsu Dock"
+ },
+ {
+ "name": "Caspataya",
+ "pos_x": 41.71875,
+ "pos_y": -179.09375,
+ "pos_z": -7.8125,
+ "stations": "Tanaka Mine"
+ },
+ {
+ "name": "Caspatha",
+ "pos_x": -164.90625,
+ "pos_y": 49.6875,
+ "pos_z": 21.46875,
+ "stations": "Margulies Ring,Schmidt Ring,Scalzi Vision,Brooks Settlement,Burckhardt Mine"
+ },
+ {
+ "name": "Caspatsuria",
+ "pos_x": 63.5,
+ "pos_y": -116.90625,
+ "pos_z": 48.15625,
+ "stations": "Meinel Orbital,Apianus Orbital,Binney Dock,Ehrenfried Kegel Prospect,van de Hulst's Progress,Sutter Arsenal"
+ },
+ {
+ "name": "Caspi",
+ "pos_x": -66.46875,
+ "pos_y": -98.125,
+ "pos_z": 10.90625,
+ "stations": "Fontana Orbital,Morukov City,Savinykh Arsenal,Smith Camp"
+ },
+ {
+ "name": "Caspian",
+ "pos_x": 20.90625,
+ "pos_y": -81.375,
+ "pos_z": -103.34375,
+ "stations": "Efremov Platform"
+ },
+ {
+ "name": "Caspiat",
+ "pos_x": 154.0625,
+ "pos_y": -212.59375,
+ "pos_z": 75.34375,
+ "stations": "McKee Orbital,Borrego Ring,Hogg Hub,Dedman Relay,Mandel Observatory"
+ },
+ {
+ "name": "Caspiatanga",
+ "pos_x": 17.03125,
+ "pos_y": 120.71875,
+ "pos_z": 18.78125,
+ "stations": "Popov Works,Cabrera Dock,Kettle Vision"
+ },
+ {
+ "name": "Cassurindui",
+ "pos_x": 151.9375,
+ "pos_y": 15.375,
+ "pos_z": -58.09375,
+ "stations": "Wisniewski-Snerg Terminal,Vela Prospect,Pond Port"
+ },
+ {
+ "name": "Catalauni",
+ "pos_x": 59.8125,
+ "pos_y": 75.21875,
+ "pos_z": -137.65625,
+ "stations": "Lander Station,Hardy Station,Yano Ring,Weyl Installation"
+ },
+ {
+ "name": "Catarihas",
+ "pos_x": 84.53125,
+ "pos_y": 7.9375,
+ "pos_z": 61.875,
+ "stations": "Springer Landing,Aleksandrov Port,Hardwick Hangar"
+ },
+ {
+ "name": "Catarites",
+ "pos_x": -99.59375,
+ "pos_y": -70.4375,
+ "pos_z": 57.5625,
+ "stations": "Artsebarsky Dock,Wiberg Installation,Teng-hui Terminal"
+ },
+ {
+ "name": "Cate",
+ "pos_x": 66.84375,
+ "pos_y": 74.46875,
+ "pos_z": -61.1875,
+ "stations": "Clifford Depot,Vela City,Haldeman II Landing,Rochon Keep"
+ },
+ {
+ "name": "Catuca",
+ "pos_x": -119.1875,
+ "pos_y": 5.09375,
+ "pos_z": 31.3125,
+ "stations": "Guin Terminal,Gloss Terminal,Clair Terminal"
+ },
+ {
+ "name": "Catucab",
+ "pos_x": 73.28125,
+ "pos_y": -235.96875,
+ "pos_z": -20,
+ "stations": "Vyssotsky Outpost"
+ },
+ {
+ "name": "Catucandit",
+ "pos_x": -82.8125,
+ "pos_y": 80.8125,
+ "pos_z": -80.9375,
+ "stations": "Galiano Station,Cook Hub,Oltion City,Kent Hub,Barentsz Ring,Werber Port,Bottego Hub,Pytheas Terminal,Binder Survey,RenenBellot Point,Macgregor Installation,Effinger Depot"
+ },
+ {
+ "name": "Catucaya",
+ "pos_x": -72.6875,
+ "pos_y": -43.4375,
+ "pos_z": 26.8125,
+ "stations": "McDivitt City"
+ },
+ {
+ "name": "Catun",
+ "pos_x": -4.71875,
+ "pos_y": 25.625,
+ "pos_z": -105.0625,
+ "stations": "Gardner Orbital,Dirichlet Arsenal"
+ },
+ {
+ "name": "Catuncheim",
+ "pos_x": -133.4375,
+ "pos_y": -7.0625,
+ "pos_z": -18.90625,
+ "stations": "Daniel Depot,Stephens' Progress,Gabriel Sanctuary,Remec Refinery"
+ },
+ {
+ "name": "Catuntinigi",
+ "pos_x": -52.65625,
+ "pos_y": 30.78125,
+ "pos_z": -51.90625,
+ "stations": "Loncke Hub,Hui Enterprise,Qian Installation"
+ },
+ {
+ "name": "Caturi",
+ "pos_x": 87.96875,
+ "pos_y": -130.40625,
+ "pos_z": 130.28125,
+ "stations": "Stiles Port,Lomonosov Colony,Cantor Prospect"
+ },
+ {
+ "name": "Cauac",
+ "pos_x": 0.21875,
+ "pos_y": 60.9375,
+ "pos_z": -80.15625,
+ "stations": "McCandless Station,Walker Platform,Lazutkin Gateway,Grigson Silo,Priest's Claim"
+ },
+ {
+ "name": "Cauan Tzu",
+ "pos_x": -172.5,
+ "pos_y": 48.34375,
+ "pos_z": -100.9375,
+ "stations": "Slusser Mines,Vonnegut's Folly"
+ },
+ {
+ "name": "Cauani",
+ "pos_x": -60.78125,
+ "pos_y": 18.78125,
+ "pos_z": 74.375,
+ "stations": "Bentham Terminal,Harrison Hub,Woolley Orbital,Hardy City,Laval Horizons,Eilenberg Port,Poisson Hub,Bohr Vision,Hedley Hub,Napier Orbital"
+ },
+ {
+ "name": "Caucan",
+ "pos_x": -27.3125,
+ "pos_y": 2.5,
+ "pos_z": 7.5625,
+ "stations": "Morukov Settlement,Culpeper Station"
+ },
+ {
+ "name": "Caucandaga",
+ "pos_x": 64.125,
+ "pos_y": 58.09375,
+ "pos_z": -91.65625,
+ "stations": "Scobee Orbital,Brunel Dock,Popper's Folly"
+ },
+ {
+ "name": "Caucanik",
+ "pos_x": -118.4375,
+ "pos_y": 40.6875,
+ "pos_z": 3.09375,
+ "stations": "Ross Point,Furukawa City,Tarentum Barracks"
+ },
+ {
+ "name": "Caucini",
+ "pos_x": 90.03125,
+ "pos_y": 3.4375,
+ "pos_z": 113.75,
+ "stations": "Williams Refinery,Kube-McDowell Installation"
+ },
+ {
+ "name": "Caucuma",
+ "pos_x": -174.875,
+ "pos_y": -10.71875,
+ "pos_z": 108.03125,
+ "stations": "Fu Station,Morris Barracks,Porges Terminal,Nourse Plant,Abel Station"
+ },
+ {
+ "name": "Cauda",
+ "pos_x": -104.21875,
+ "pos_y": -31.46875,
+ "pos_z": 58.34375,
+ "stations": "McCormick Camp,Wright Depot,Fairbairn Landing"
+ },
+ {
+ "name": "Caudjabe",
+ "pos_x": 38.5,
+ "pos_y": 38.875,
+ "pos_z": 137.78125,
+ "stations": "Malpighi Station,Macarthur Vision,Cartmill Terminal,Wisniewski-Snerg Base"
+ },
+ {
+ "name": "Caudji",
+ "pos_x": 94.4375,
+ "pos_y": -242.78125,
+ "pos_z": 83.28125,
+ "stations": "Mayer City,Schroter Port,Lopez de Haro Relay,Isherwood Survey"
+ },
+ {
+ "name": "Cava",
+ "pos_x": 76.40625,
+ "pos_y": -31.71875,
+ "pos_z": -128.1875,
+ "stations": "Soper Dock"
+ },
+ {
+ "name": "Cavane",
+ "pos_x": -16.875,
+ "pos_y": -95.21875,
+ "pos_z": 139.875,
+ "stations": "Wisdom Dock,Luiken Installation,Reichelt's Progress"
+ },
+ {
+ "name": "Cavaniaci",
+ "pos_x": -22.4375,
+ "pos_y": -159.125,
+ "pos_z": 94.59375,
+ "stations": "Dillon Platform,Hughes Ring,Oort Platform,Blaauw Penal colony"
+ },
+ {
+ "name": "Cavari",
+ "pos_x": 114.46875,
+ "pos_y": -101.09375,
+ "pos_z": -56.125,
+ "stations": "Reinmuth Terminal,Mainzer Port,Otus Station"
+ },
+ {
+ "name": "Cavincan",
+ "pos_x": 54.125,
+ "pos_y": 40.8125,
+ "pos_z": 141.53125,
+ "stations": "Stabenow Port,Cremona Terminal,Barreiros Orbital,Holden's Progress"
+ },
+ {
+ "name": "Cavins",
+ "pos_x": 27,
+ "pos_y": 8.78125,
+ "pos_z": -7.0625,
+ "stations": "Nye Port,Alcala Hub,McNair Ring"
+ },
+ {
+ "name": "Cayu",
+ "pos_x": 33.5625,
+ "pos_y": -261.5,
+ "pos_z": 35.25,
+ "stations": "Westphal Survey"
+ },
+ {
+ "name": "Cayura",
+ "pos_x": 0.15625,
+ "pos_y": -110.34375,
+ "pos_z": -15.03125,
+ "stations": "Gagarin Colony,Crampton Station,Collins Prospect"
+ },
+ {
+ "name": "Cayutorme",
+ "pos_x": 116.3125,
+ "pos_y": -76.90625,
+ "pos_z": 8.25,
+ "stations": "Adkins Port,Bretnor Observatory"
+ },
+ {
+ "name": "CC Eri",
+ "pos_x": 16.65625,
+ "pos_y": -33.8125,
+ "pos_z": -3.65625,
+ "stations": "Gooch Terminal,Hire Port,Kuttner Silo"
+ },
+ {
+ "name": "CD-22 12617",
+ "pos_x": -16.46875,
+ "pos_y": -3.40625,
+ "pos_z": 105.625,
+ "stations": "Mendeleev Orbital,Barsanti Market,Silves Base,Warner Hub,Macan Vista,Cori Terminal,Anvil Base,Eschbach Observatory"
+ },
+ {
+ "name": "CD-22 9062",
+ "pos_x": 89.90625,
+ "pos_y": 69.34375,
+ "pos_z": 21.6875,
+ "stations": "Noriega Dock,Helms Terminal,Bowen Market,Macarthur Dock,Roberts Penal colony,Pythagoras City,Hertz Works,Crippen Station,Horch Dock,Sarmiento de Gamboa's Progress,Hewish Prospect"
+ },
+ {
+ "name": "CD-24 10236",
+ "pos_x": 70,
+ "pos_y": 57.75,
+ "pos_z": 30.03125,
+ "stations": "Scully-Power Dock"
+ },
+ {
+ "name": "CD-24 10619",
+ "pos_x": 65.5625,
+ "pos_y": 61.84375,
+ "pos_z": 46.15625,
+ "stations": "Kennedy Hub,Mieville Terminal,Kennan Orbital,Lawhead Installation,O'Brien Bastion"
+ },
+ {
+ "name": "CD-24 5005",
+ "pos_x": 73.125,
+ "pos_y": -9.84375,
+ "pos_z": -46.1875,
+ "stations": "Hoshi Port,McAuley Dock"
+ },
+ {
+ "name": "CD-25 1159",
+ "pos_x": 26.28125,
+ "pos_y": -83.25,
+ "pos_z": -36.375,
+ "stations": "Alexandrov Landing,Paulmier de Gonneville Landing,Williams Orbital"
+ },
+ {
+ "name": "CD-25 636",
+ "pos_x": 7,
+ "pos_y": -107,
+ "pos_z": -17.96875,
+ "stations": "Fieseler Dock,Goonan's Folly,Bolkow Ring,Luu Keep"
+ },
+ {
+ "name": "CD-26 14379",
+ "pos_x": -26.21875,
+ "pos_y": -42.78125,
+ "pos_z": 103.03125,
+ "stations": "Frimout Terminal,Richards Dock,Skvortsov Station"
+ },
+ {
+ "name": "CD-26 369",
+ "pos_x": 2.40625,
+ "pos_y": -114.53125,
+ "pos_z": -9.0625,
+ "stations": "Xuesen Estate,Truman Holdings,Jean Settlement,Gurney Vision,Gent Refinery,Forstchen Landing"
+ },
+ {
+ "name": "CD-27 10200",
+ "pos_x": 40.90625,
+ "pos_y": 47.90625,
+ "pos_z": 91.65625,
+ "stations": "Hedin Settlement,Flynn Prospect,Shipton Base"
+ },
+ {
+ "name": "CD-27 2736",
+ "pos_x": 79.375,
+ "pos_y": -37.78125,
+ "pos_z": -56.875,
+ "stations": "Neff Prospect"
+ },
+ {
+ "name": "CD-27 5409",
+ "pos_x": 100.09375,
+ "pos_y": 10.78125,
+ "pos_z": -39.5,
+ "stations": "Bellamy Dock,al-Haytham Settlement,Plexico Dock,Bunch Beacon,Howard Orbital,Raleigh Port,Adams Gateway,Aitken City,Meaney Dock,West City,Shipton Terminal"
+ },
+ {
+ "name": "CD-28 12950",
+ "pos_x": 6.21875,
+ "pos_y": 10.1875,
+ "pos_z": 110.71875,
+ "stations": "Keyes Port,Hovell City,VanderMeer Ring,Besonders Terminal"
+ },
+ {
+ "name": "CD-28 18350",
+ "pos_x": -12.3125,
+ "pos_y": -112.0625,
+ "pos_z": 23.75,
+ "stations": "Oikawa City,Morrill Station,Asclepi Terminal,Sternbach Holdings"
+ },
+ {
+ "name": "CD-28 18361",
+ "pos_x": -11.75,
+ "pos_y": -108.03125,
+ "pos_z": 22.46875,
+ "stations": "Euclid Hub,Reynolds Orbital,Dickson Installation,Mukai Hub,Burton Vista"
+ },
+ {
+ "name": "CD-30 11949",
+ "pos_x": 44.6875,
+ "pos_y": 45.65625,
+ "pos_z": 95,
+ "stations": "Herbert Co-operative,Brooks Lab,Schroeder Silo"
+ },
+ {
+ "name": "CD-30 9687",
+ "pos_x": 86.21875,
+ "pos_y": 54.78125,
+ "pos_z": 36.15625,
+ "stations": "Savitskaya Ring,Wingrove Arsenal"
+ },
+ {
+ "name": "CD-31 1974",
+ "pos_x": 58.75,
+ "pos_y": -62.75,
+ "pos_z": -46.09375,
+ "stations": "Wachmann Port"
+ },
+ {
+ "name": "CD-31 2366",
+ "pos_x": 81.875,
+ "pos_y": -62.25,
+ "pos_z": -58.625,
+ "stations": "Altuna City,Lippisch Orbital,Lintott City,Bohrmann Vision,Lloyd Wright Station,Baade Station,Lavochkin City,Hay Depot,Brundage Hub,Stevens Base"
+ },
+ {
+ "name": "CD-32 11850",
+ "pos_x": 17.4375,
+ "pos_y": 13.28125,
+ "pos_z": 84.40625,
+ "stations": "Anvil Survey"
+ },
+ {
+ "name": "CD-33 1835",
+ "pos_x": 70.28125,
+ "pos_y": -74.84375,
+ "pos_z": -49.875,
+ "stations": "Oleskiw City,Descartes Station"
+ },
+ {
+ "name": "CD-33 8748",
+ "pos_x": 46.21875,
+ "pos_y": 30.15625,
+ "pos_z": 33.15625,
+ "stations": "Viktorenko Holdings"
+ },
+ {
+ "name": "CD-34 13042",
+ "pos_x": -2,
+ "pos_y": -26.4375,
+ "pos_z": 107.6875,
+ "stations": "Thomson Relay,Iqbal Horizons"
+ },
+ {
+ "name": "CD-34 9020",
+ "pos_x": 71.28125,
+ "pos_y": 49.46875,
+ "pos_z": 69.15625,
+ "stations": "Back Enterprise,Daimler Town"
+ },
+ {
+ "name": "CD-35 12664",
+ "pos_x": 2.40625,
+ "pos_y": -24.53125,
+ "pos_z": 113.59375,
+ "stations": "Crown Estate,Baudin Prospect,Temple Barracks,Herreshoff Estate"
+ },
+ {
+ "name": "CD-35 14353",
+ "pos_x": -13.6875,
+ "pos_y": -74.5,
+ "pos_z": 92.46875,
+ "stations": "Viehbock Dock,Tognini Stop,Howard Survey"
+ },
+ {
+ "name": "CD-35 6972",
+ "pos_x": 81.71875,
+ "pos_y": 34.21875,
+ "pos_z": 15.375,
+ "stations": "Napier's Folly,Papin Terminal"
+ },
+ {
+ "name": "CD-35 9019",
+ "pos_x": 59.875,
+ "pos_y": 41.125,
+ "pos_z": 62.9375,
+ "stations": "Skvortsov Holdings,Liska Port,Liwei Orbital,Brahe Gateway,Clark Station,Scobee Terminal,Ham Terminal,Wright City,Beadle Terminal,Baudry Vision,Kraepelin Orbital,Pelt Base"
+ },
+ {
+ "name": "CD-36 12714",
+ "pos_x": 2.8125,
+ "pos_y": -20.53125,
+ "pos_z": 92.75,
+ "stations": "Westerfeld Prospect,Gilliland Dock,Farrukh Terminal,Trinh Colony,Gallun Terminal,MacDonald Ring,Mallett Station,Pook Reach,Garfinkle Market"
+ },
+ {
+ "name": "CD-36 1535",
+ "pos_x": 65.15625,
+ "pos_y": -89.625,
+ "pos_z": -41.09375,
+ "stations": "Aoki Hub,Forfait Installation"
+ },
+ {
+ "name": "CD-36 1916",
+ "pos_x": 66.75,
+ "pos_y": -62.1875,
+ "pos_z": -40.9375,
+ "stations": "Romanenko Port"
+ },
+ {
+ "name": "CD-36 874",
+ "pos_x": 34.59375,
+ "pos_y": -103.34375,
+ "pos_z": -19,
+ "stations": "Denton Platform,Konscak Depot"
+ },
+ {
+ "name": "CD-37 1883",
+ "pos_x": 68.09375,
+ "pos_y": -66.15625,
+ "pos_z": -39.5,
+ "stations": "Savitskaya Ring"
+ },
+ {
+ "name": "CD-37 641",
+ "pos_x": 27.65625,
+ "pos_y": -112.75,
+ "pos_z": -6.9375,
+ "stations": "Froud Hub,Napier Legacy,Lagrange City,Tikhonravov City,Ball Dock,Dantec Palace,Qureshi Dock,Hall Hub"
+ },
+ {
+ "name": "CD-39 14212",
+ "pos_x": -6.125,
+ "pos_y": -81.5625,
+ "pos_z": 79.75,
+ "stations": "Whitson Port,Rennie Port,Wisoff Terminal,Rothfuss Base,Bushnell Dock,Clement Installation,Carver Enterprise,Galiano Beacon,Nelson Vision,Hernandez's Inheritance,Kornbluth Reach"
+ },
+ {
+ "name": "CD-39 3269",
+ "pos_x": 110.53125,
+ "pos_y": -22.75,
+ "pos_z": -36.625,
+ "stations": "Shelley Station,Sturt's Inheritance,Fiennes Gateway,Jacobi City,Lloyd Ring,Saavedra City,McDevitt Station,Onufriyenko Legacy,Oxley Depot,Reynolds Hub,Nansen Settlement,Schomburg Depot"
+ },
+ {
+ "name": "CD-39 4830",
+ "pos_x": 114.40625,
+ "pos_y": 5.1875,
+ "pos_z": -17.40625,
+ "stations": "Thomas City,Matteucci Ring,Person Landing,Stevin Lab,Green's Progress,MacLean Penal colony,Peral Arena"
+ },
+ {
+ "name": "CD-40 395",
+ "pos_x": 34,
+ "pos_y": -119.8125,
+ "pos_z": -1.03125,
+ "stations": "Oort Port,Merritt Dock,Powers Landing"
+ },
+ {
+ "name": "CD-41 359",
+ "pos_x": 45.5625,
+ "pos_y": -165.125,
+ "pos_z": 6.46875,
+ "stations": "May Vision"
+ },
+ {
+ "name": "CD-41 568",
+ "pos_x": 36.03125,
+ "pos_y": -99,
+ "pos_z": -6.65625,
+ "stations": "Gent Vision,Russell Bastion,Allen Relay"
+ },
+ {
+ "name": "CD-43 11917",
+ "pos_x": 18,
+ "pos_y": -10.5625,
+ "pos_z": 78.125,
+ "stations": "Attilius Orbital"
+ },
+ {
+ "name": "CD-43 9401",
+ "pos_x": 60.65625,
+ "pos_y": 25.375,
+ "pos_z": 87.75,
+ "stations": "Seddon Legacy,Eyharts Retreat"
+ },
+ {
+ "name": "CD-44 10336",
+ "pos_x": 53.34375,
+ "pos_y": 15.59375,
+ "pos_z": 102.9375,
+ "stations": "Margulis Station,Herschel Horizons,Maxwell Ring"
+ },
+ {
+ "name": "CD-44 224",
+ "pos_x": 38.375,
+ "pos_y": -154.90625,
+ "pos_z": 23.4375,
+ "stations": "Koolhaas Vision"
+ },
+ {
+ "name": "CD-45 11273",
+ "pos_x": 33.3125,
+ "pos_y": -8.5,
+ "pos_z": 105.875,
+ "stations": "Asher Orbital"
+ },
+ {
+ "name": "CD-45 7854",
+ "pos_x": 88.53125,
+ "pos_y": 29.46875,
+ "pos_z": 51.90625,
+ "stations": "Dana Mines,Onufrienko Oasis"
+ },
+ {
+ "name": "CD-45 973",
+ "pos_x": 65.1875,
+ "pos_y": -89.4375,
+ "pos_z": 1.53125,
+ "stations": "Gotz Prospect"
+ },
+ {
+ "name": "CD-46 12902",
+ "pos_x": 6.59375,
+ "pos_y": -21.28125,
+ "pos_z": 47.53125,
+ "stations": "Searfoss Orbital"
+ },
+ {
+ "name": "CD-46 14401",
+ "pos_x": 8.125,
+ "pos_y": -62.625,
+ "pos_z": 37.375,
+ "stations": "Bendell Gateway,Klein Enterprise,Stith Ring"
+ },
+ {
+ "name": "CD-47 8991",
+ "pos_x": 76.53125,
+ "pos_y": 24.15625,
+ "pos_z": 83.1875,
+ "stations": "Blodgett Gateway,Crown Holdings"
+ },
+ {
+ "name": "CD-47 990",
+ "pos_x": 68.96875,
+ "pos_y": -103.40625,
+ "pos_z": -14.84375,
+ "stations": "Nilson Installation,Druillet Station,Pogson City,Jackson Ring,Mitchison Installation"
+ },
+ {
+ "name": "CD-48 3774",
+ "pos_x": 107.90625,
+ "pos_y": -12.1875,
+ "pos_z": -7.46875,
+ "stations": "Panshin Ring"
+ },
+ {
+ "name": "CD-49 13082",
+ "pos_x": 15.84375,
+ "pos_y": -62.6875,
+ "pos_z": 87,
+ "stations": "Marius Relay,Theodorsen Mines"
+ },
+ {
+ "name": "CD-49 1643",
+ "pos_x": 84.84375,
+ "pos_y": -62,
+ "pos_z": -21.21875,
+ "stations": "Piaget Horizons"
+ },
+ {
+ "name": "CD-49 3617",
+ "pos_x": 140.8125,
+ "pos_y": -15.375,
+ "pos_z": -6.75,
+ "stations": "Fife Horizons,Rasch Ring,Kazantsev Prospect,Jeans Lab"
+ },
+ {
+ "name": "CD-51 102",
+ "pos_x": 40.71875,
+ "pos_y": -124.15625,
+ "pos_z": 36.78125,
+ "stations": "McDermott Enterprise,McMullen Penal colony"
+ },
+ {
+ "name": "CD-51 1447",
+ "pos_x": 95.59375,
+ "pos_y": -68.21875,
+ "pos_z": -19.625,
+ "stations": "Hell Orbital,Giclas Orbital,Stromgren Terminal,Faber Hub,Knorre Port,Campbell Orbital,Malzberg Gateway,Frimout Station,Evans Gateway"
+ },
+ {
+ "name": "CD-51 2650",
+ "pos_x": 95.25,
+ "pos_y": -23.1875,
+ "pos_z": -8.09375,
+ "stations": "Walker Platform,Noether Dock,Clough Base,Brust Prospect"
+ },
+ {
+ "name": "CD-51 4783",
+ "pos_x": 107.0625,
+ "pos_y": 8.3125,
+ "pos_z": 23.59375,
+ "stations": "Al Saud Stop,Hilbert Installation"
+ },
+ {
+ "name": "CD-51 881",
+ "pos_x": 75.75,
+ "pos_y": -90.53125,
+ "pos_z": -11.125,
+ "stations": "Sukhoi Installation,Potez Station,Hoerner City,Corte-Real Reach,Mayer Works"
+ },
+ {
+ "name": "CD-51 9202",
+ "pos_x": 62.21875,
+ "pos_y": 5.125,
+ "pos_z": 94.375,
+ "stations": "Bond Enterprise,Pellegrino Holdings,Holdstock City,Rucker Base"
+ },
+ {
+ "name": "CD-52 187",
+ "pos_x": 33.8125,
+ "pos_y": -86.6875,
+ "pos_z": 19.40625,
+ "stations": "Schoenherr Station,Lomonosov Ring,Sopwith Port,Nojiri Dock,Morrow Ring,Younghusband Exchange,Barmin City,Cummings Dock,Hynek City,Lawhead Legacy,Lowell Vision"
+ },
+ {
+ "name": "CD-52 557",
+ "pos_x": 80.6875,
+ "pos_y": -125.34375,
+ "pos_z": -0.0625,
+ "stations": "Sewell Port"
+ },
+ {
+ "name": "CD-52 9466",
+ "pos_x": 14.21875,
+ "pos_y": -45.15625,
+ "pos_z": 62.375,
+ "stations": "Jakes Arsenal,Miklouho-Maclay Station,Makeev Platform"
+ },
+ {
+ "name": "CD-52 9732",
+ "pos_x": 17.4375,
+ "pos_y": -63.84375,
+ "pos_z": 70.03125,
+ "stations": "Roberts Port,Dyr Terminal"
+ },
+ {
+ "name": "CD-53 136",
+ "pos_x": 51.71875,
+ "pos_y": -131.625,
+ "pos_z": 37.28125,
+ "stations": "Lyne Holdings,Bauschinger Settlement,Thesiger Plant,Schuster Dock,Theodor Winnecke Station,Watts Base"
+ },
+ {
+ "name": "CD-53 9442",
+ "pos_x": 43.5625,
+ "pos_y": -136.15625,
+ "pos_z": 56.09375,
+ "stations": "Folland Station,Roemer Dock,Vuia Beacon,Dunlop Dock,Ulloa Base"
+ },
+ {
+ "name": "CD-54 471",
+ "pos_x": 46.90625,
+ "pos_y": -80.5,
+ "pos_z": 6.9375,
+ "stations": "KRAMSKI Holding,Cochrane Platform"
+ },
+ {
+ "name": "CD-54 4840",
+ "pos_x": 101.9375,
+ "pos_y": 15.84375,
+ "pos_z": 60.71875,
+ "stations": "Garriott Enterprise,Holland Survey,Poisson Beacon"
+ },
+ {
+ "name": "CD-54 9671",
+ "pos_x": 33.9375,
+ "pos_y": -97.34375,
+ "pos_z": 39.03125,
+ "stations": "Somayaji Terminal,Tshang Relay,Mohr Landing"
+ },
+ {
+ "name": "CD-55 1514",
+ "pos_x": 39.59375,
+ "pos_y": -17.375,
+ "pos_z": -3.5,
+ "stations": "Laveykin Port,Kendrick Hub,Dirac Ring,Hardwick Hub,Hiraga Holdings,Ray Holdings,Edgeworth Terminal"
+ },
+ {
+ "name": "CD-55 8747",
+ "pos_x": 24.90625,
+ "pos_y": -76.3125,
+ "pos_z": 80.09375,
+ "stations": "Wright Dock,Qian Works,Perrine Colony,Bauman Colony"
+ },
+ {
+ "name": "CD-58 4207",
+ "pos_x": 117.4375,
+ "pos_y": 6.46875,
+ "pos_z": 54.09375,
+ "stations": "Sarmiento de Gamboa Ring,Cartmill Port"
+ },
+ {
+ "name": "CD-58 538",
+ "pos_x": 32.125,
+ "pos_y": -44.3125,
+ "pos_z": 4.90625,
+ "stations": "Meade Ring,Hornby Station,Haberlandt Orbital"
+ },
+ {
+ "name": "CD-58 7880",
+ "pos_x": 32.78125,
+ "pos_y": -85.40625,
+ "pos_z": 76.78125,
+ "stations": "Wolf Dock,Zeppelin City,Zhuravleva City,Wagner Landing,Fanning's Progress"
+ },
+ {
+ "name": "CD-59 1681",
+ "pos_x": 102.3125,
+ "pos_y": -31.125,
+ "pos_z": 3.0625,
+ "stations": "Bobko Mines,Scully-Power Survey,McQuay Penal colony"
+ },
+ {
+ "name": "CD-59 1724",
+ "pos_x": 104.3125,
+ "pos_y": -30.25,
+ "pos_z": 4,
+ "stations": "Herbert Refinery,Velazquez Observatory"
+ },
+ {
+ "name": "CD-59 4616",
+ "pos_x": 79.5,
+ "pos_y": 3.875,
+ "pos_z": 57.5625,
+ "stations": "Tsunenaga Base,Vinogradov Colony"
+ },
+ {
+ "name": "CD-59 7632",
+ "pos_x": 31.78125,
+ "pos_y": -69.6875,
+ "pos_z": 74.875,
+ "stations": "Kuhn Dock,Watson Beacon"
+ },
+ {
+ "name": "CD-60 278",
+ "pos_x": 89.875,
+ "pos_y": -153.875,
+ "pos_z": 40.875,
+ "stations": "Chacornac's Progress,McManus Barracks"
+ },
+ {
+ "name": "CD-60 31",
+ "pos_x": 63.09375,
+ "pos_y": -127.375,
+ "pos_z": 53.40625,
+ "stations": "Arkhangelsky Retreat,Kirk Penal colony"
+ },
+ {
+ "name": "CD-60 7742",
+ "pos_x": 37.65625,
+ "pos_y": -81.5,
+ "pos_z": 75.875,
+ "stations": "Kopal Dock,Friedman Ring,Bulgakov Camp,Lethem Base"
+ },
+ {
+ "name": "CD-61 1439",
+ "pos_x": 66.09375,
+ "pos_y": -30.71875,
+ "pos_z": 1.4375,
+ "stations": "Whitney Station,Wedge Port,Bowen Market,Huygens Gateway"
+ },
+ {
+ "name": "CD-61 6651",
+ "pos_x": 25.53125,
+ "pos_y": -55.15625,
+ "pos_z": 46.4375,
+ "stations": "Crouch Orbital,Walheim City,Oswald Hub,Polansky's Inheritance"
+ },
+ {
+ "name": "CD-61 6801",
+ "pos_x": 41.5,
+ "pos_y": -86.96875,
+ "pos_z": 50.90625,
+ "stations": "Bus Port,Gamow Bastion,Nagata Enterprise"
+ },
+ {
+ "name": "CD-62 136",
+ "pos_x": 109.53125,
+ "pos_y": -115.625,
+ "pos_z": 13.09375,
+ "stations": "Duque Gateway,Bradley Horizons,Apianus Dock,Reichelt Vision,Wu Base,Naburimannu Orbital,Bennett Gateway,Sagan Port"
+ },
+ {
+ "name": "CD-62 1454",
+ "pos_x": 50.9375,
+ "pos_y": -103.59375,
+ "pos_z": 56.25,
+ "stations": "Baille City,Westphal Dock,Patry Station"
+ },
+ {
+ "name": "CD-62 234",
+ "pos_x": 76.34375,
+ "pos_y": -42.25,
+ "pos_z": 2.625,
+ "stations": "Menezes Horizons,Burton Barracks,Vyssotsky Lab"
+ },
+ {
+ "name": "CD-63 1560",
+ "pos_x": 42.375,
+ "pos_y": -85.09375,
+ "pos_z": 64.15625,
+ "stations": "Barmin Ring,Watanabe City,Taylor Vision,Montanari Arsenal"
+ },
+ {
+ "name": "CD-63 201",
+ "pos_x": 122.5625,
+ "pos_y": -81.1875,
+ "pos_z": 5.53125,
+ "stations": "Szentmartony City,VanderMeer Palace,Dashiell Orbital,Bernoulli Gateway,Savorgnan de Brazza Works,Clapperton Enterprise,Derleth Landing,Nourse Dock,Lorrah City,Nicolet Vision,Cartmill Terminal"
+ },
+ {
+ "name": "CD-63 359",
+ "pos_x": 109.875,
+ "pos_y": -35.125,
+ "pos_z": 12.375,
+ "stations": "Benz Point"
+ },
+ {
+ "name": "CD-64 139",
+ "pos_x": 87.125,
+ "pos_y": -139.1875,
+ "pos_z": 56.03125,
+ "stations": "Pook Silo,Christa McAuliffe,Guerrero Installation"
+ },
+ {
+ "name": "CD-65 76",
+ "pos_x": 89.75,
+ "pos_y": -124.28125,
+ "pos_z": 42.25,
+ "stations": "Jack E. Stuart Memorial Spaceport,Alex's Rest"
+ },
+ {
+ "name": "CD-66 2585",
+ "pos_x": 54.4375,
+ "pos_y": -93.3125,
+ "pos_z": 65.9375,
+ "stations": "Witt Terminal,Dahm City,Blish Prospect,Comper Survey"
+ },
+ {
+ "name": "CD-67 2611",
+ "pos_x": 71.625,
+ "pos_y": -114.3125,
+ "pos_z": 65.21875,
+ "stations": "Robert Mines,Pritchard Settlement,Pilyugin Relay"
+ },
+ {
+ "name": "CD-68 2054",
+ "pos_x": 56.03125,
+ "pos_y": -53.625,
+ "pos_z": 87.84375,
+ "stations": "Hereford Settlement"
+ },
+ {
+ "name": "CD-68 29",
+ "pos_x": 86.4375,
+ "pos_y": -121.46875,
+ "pos_z": 57.15625,
+ "stations": "Truman Station"
+ },
+ {
+ "name": "CD-69 5",
+ "pos_x": 76.90625,
+ "pos_y": -110.09375,
+ "pos_z": 59.375,
+ "stations": "Clements Sentinel Industries,Williamson Refinery,Samphire's Solace,Dias Platform"
+ },
+ {
+ "name": "CD-69 55",
+ "pos_x": 105.90625,
+ "pos_y": -135.5,
+ "pos_z": 58.34375,
+ "stations": "Gaultier de Varennes Point"
+ },
+ {
+ "name": "CD-70 19",
+ "pos_x": 108.84375,
+ "pos_y": -143.96875,
+ "pos_z": 78.25,
+ "stations": "Beriev Stop,Verrier Survey,Mori City,Rich Depot"
+ },
+ {
+ "name": "CD-70 1960",
+ "pos_x": 75.84375,
+ "pos_y": -102.8125,
+ "pos_z": 60.125,
+ "stations": "Marc Palmans,Ross Depot,Brodie's Pride"
+ },
+ {
+ "name": "CD-72 1783",
+ "pos_x": 73.5625,
+ "pos_y": -94.5625,
+ "pos_z": 65.8125,
+ "stations": "Flint Keep,Hirn Horizons,Lubbock Survey"
+ },
+ {
+ "name": "CD-72 190",
+ "pos_x": 113.0625,
+ "pos_y": -113.4375,
+ "pos_z": 47.0625,
+ "stations": "Phillips Terminal,Bennett Orbital,Karman Orbital"
+ },
+ {
+ "name": "CD-73 12",
+ "pos_x": 82.9375,
+ "pos_y": -99.4375,
+ "pos_z": 59.5625,
+ "stations": "Barbuy Penal colony,Mysh Mad,Alexander Brongniart"
+ },
+ {
+ "name": "CD-73 253",
+ "pos_x": 103.28125,
+ "pos_y": -64.84375,
+ "pos_z": 27.28125,
+ "stations": "Robinson Station"
+ },
+ {
+ "name": "CD-74 1370",
+ "pos_x": 63.4375,
+ "pos_y": -61.71875,
+ "pos_z": 78.625,
+ "stations": "Wenzel Port"
+ },
+ {
+ "name": "CD-74 144",
+ "pos_x": 123.90625,
+ "pos_y": -110.625,
+ "pos_z": 49.09375,
+ "stations": "Johnson Installation,Elst Hub"
+ },
+ {
+ "name": "CD-74 632",
+ "pos_x": 95.84375,
+ "pos_y": -25.625,
+ "pos_z": 52.4375,
+ "stations": "Efremov Enterprise,Brooks' Progress,RenenBellot Hub,Simmons Port,Tayler Orbital,Clebsch Terminal,Bester Works,Blair Prospect,Paez Stop,Crouch Laboratory,Citi Installation"
+ },
+ {
+ "name": "CD-75 661",
+ "pos_x": 67.875,
+ "pos_y": -21.5,
+ "pos_z": 51.15625,
+ "stations": "Kirk Dock,Neujmin Station"
+ },
+ {
+ "name": "CD-76 1091",
+ "pos_x": 59.25,
+ "pos_y": -62.53125,
+ "pos_z": 63.4375,
+ "stations": "Altman Terminal"
+ },
+ {
+ "name": "CD-77 45",
+ "pos_x": 99.25,
+ "pos_y": -98.6875,
+ "pos_z": 58.1875,
+ "stations": "Irrelon Orbital,Delsanti Station"
+ },
+ {
+ "name": "CD-79 950",
+ "pos_x": 87.96875,
+ "pos_y": -85.09375,
+ "pos_z": 63.6875,
+ "stations": "Emma Jayne Wells Orbital,Alex Prior High,Hannu Mines,Trumpler Survey"
+ },
+ {
+ "name": "CD-82 331",
+ "pos_x": 68.03125,
+ "pos_y": -52.5625,
+ "pos_z": 60.40625,
+ "stations": "Lee Dock,Bates Arsenal,Rawat Dock"
+ },
+ {
+ "name": "CD-86 4",
+ "pos_x": 86.75,
+ "pos_y": -64.78125,
+ "pos_z": 56.84375,
+ "stations": "Cummings Dock,Abe Relay"
+ },
+ {
+ "name": "CD-86 84",
+ "pos_x": 91.78125,
+ "pos_y": -50.34375,
+ "pos_z": 60.1875,
+ "stations": "Mitchison Vision,Wheeler Survey,Lagerkvist Terminal,Dashiell Hub"
+ },
+ {
+ "name": "CD-87 103",
+ "pos_x": 76.5,
+ "pos_y": -52.65625,
+ "pos_z": 54.84375,
+ "stations": "Shajn City"
+ },
+ {
+ "name": "CE Bootis",
+ "pos_x": -5.40625,
+ "pos_y": 29.375,
+ "pos_z": 16.46875,
+ "stations": "Asher Gateway,Moskowitz Gateway,Hovell Terminal"
+ },
+ {
+ "name": "Cebalrai",
+ "pos_x": -38.625,
+ "pos_y": 23.40625,
+ "pos_z": 68.25,
+ "stations": "Kuhn City,Dutton Terminal,Hornblower Dock,Matthews Barracks,Blackwell Vision"
+ },
+ {
+ "name": "Ceginus",
+ "pos_x": -92.1875,
+ "pos_y": 135.59375,
+ "pos_z": 43.71875,
+ "stations": "Bunnell Platform"
+ },
+ {
+ "name": "Cegreeth",
+ "pos_x": 62.71875,
+ "pos_y": -109.0625,
+ "pos_z": 50.3125,
+ "stations": "Shaw's Inheritance,V1G Apology,Battani Dock,Volta Orbital,Hubble Vision"
+ },
+ {
+ "name": "Celaeno",
+ "pos_x": -81.09375,
+ "pos_y": -148.3125,
+ "pos_z": -337.09375,
+ "stations": "Artemis Lodge,Rescue Ship - Artemis Lodge"
+ },
+ {
+ "name": "Celeng Gu",
+ "pos_x": -1.875,
+ "pos_y": 80.40625,
+ "pos_z": -90.75,
+ "stations": "Broglie Terminal"
+ },
+ {
+ "name": "Celer",
+ "pos_x": 89.90625,
+ "pos_y": 119.46875,
+ "pos_z": -33.09375,
+ "stations": "Broglie Dock,Turtledove Point"
+ },
+ {
+ "name": "Celes",
+ "pos_x": 79.9375,
+ "pos_y": -7.625,
+ "pos_z": -149.15625,
+ "stations": "Oxley Hub,Erikson's Inheritance"
+ },
+ {
+ "name": "Celesat",
+ "pos_x": 1.4375,
+ "pos_y": 1.84375,
+ "pos_z": 140.625,
+ "stations": "Narbeth Penal colony,Shalatula Depot,Napier's Progress"
+ },
+ {
+ "name": "Celetae",
+ "pos_x": 95.375,
+ "pos_y": -42.1875,
+ "pos_z": -70.3125,
+ "stations": "Roggeveen Settlement"
+ },
+ {
+ "name": "Celevik",
+ "pos_x": -105.96875,
+ "pos_y": -85.125,
+ "pos_z": 37.875,
+ "stations": "Clervoy Orbital,Dirac Dock"
+ },
+ {
+ "name": "Celtani",
+ "pos_x": 107.78125,
+ "pos_y": -10.53125,
+ "pos_z": -84.375,
+ "stations": "Forrester Port,Yurchikhin Terminal,Carpenter Colony,Rolland Vision"
+ },
+ {
+ "name": "Celtaurii",
+ "pos_x": -97.46875,
+ "pos_y": 39.78125,
+ "pos_z": 91.75,
+ "stations": "Panshin Base,Spinrad Relay,King Outpost"
+ },
+ {
+ "name": "Celtibia",
+ "pos_x": 95.46875,
+ "pos_y": -64.71875,
+ "pos_z": 51.78125,
+ "stations": "Sabine Vision"
+ },
+ {
+ "name": "Celtibitou",
+ "pos_x": 144.875,
+ "pos_y": -17.5625,
+ "pos_z": -48.5625,
+ "stations": "Cogswell Outpost,Shaara Hangar,Witt Escape"
+ },
+ {
+ "name": "Celticassag",
+ "pos_x": -20.4375,
+ "pos_y": 42.75,
+ "pos_z": -124.0625,
+ "stations": "Burton Freeport,Porges Freeport"
+ },
+ {
+ "name": "Celtici",
+ "pos_x": 214.9375,
+ "pos_y": -146.875,
+ "pos_z": 73.1875,
+ "stations": "Jefferies Vision,Biruni Port"
+ },
+ {
+ "name": "Cemiess",
+ "pos_x": 66.0625,
+ "pos_y": -105.34375,
+ "pos_z": 27.09375,
+ "stations": "Mackenzie Relay,Jones Hub,Hamuy Port,Meech Dock,Titius Station,Pelliot Town,Shoemaker City,Glass City,Metcalf Orbital"
+ },
+ {
+ "name": "Cemplangpa",
+ "pos_x": 26.0625,
+ "pos_y": -165.75,
+ "pos_z": -9.40625,
+ "stations": "Nicholson Orbital,McQuarrie Gateway,Gorgani Orbital,Kirby City,Artzybasheff Prospect,Mather Vision,Budrys' Inheritance,Ahnert Dock,Nakamura Hub,Westphal Landing,Sohl Horizons,Heck Refinery,Mitra Silo"
+ },
+ {
+ "name": "Cemplatui",
+ "pos_x": -20.4375,
+ "pos_y": -18.1875,
+ "pos_z": -99.28125,
+ "stations": "Mallett Dock,Budrys Base,Lanier Platform,Bass Laboratory"
+ },
+ {
+ "name": "Cempsigi",
+ "pos_x": -98.96875,
+ "pos_y": -20.3125,
+ "pos_z": -25.5,
+ "stations": "Nikolayev Prospect,Flint Terminal,Wagner Hub,Schiltberger Terminal,Macan Orbital"
+ },
+ {
+ "name": "Cenil",
+ "pos_x": 55.84375,
+ "pos_y": 45.4375,
+ "pos_z": -26.53125,
+ "stations": "Helms Port,Feoktistov City"
+ },
+ {
+ "name": "Cenni",
+ "pos_x": 32.0625,
+ "pos_y": -214.71875,
+ "pos_z": 117.53125,
+ "stations": "Richer Hub,Fabian Platform"
+ },
+ {
+ "name": "Cennicenino",
+ "pos_x": 128.53125,
+ "pos_y": -4.09375,
+ "pos_z": -54.75,
+ "stations": "Stevin Market,Zahn Enterprise,Morrison Prospect,Banks Terminal"
+ },
+ {
+ "name": "Cenoi",
+ "pos_x": -74.25,
+ "pos_y": -5.65625,
+ "pos_z": -71.125,
+ "stations": "Weber Refinery"
+ },
+ {
+ "name": "Centralis",
+ "pos_x": -9516.15625,
+ "pos_y": -924.5,
+ "pos_z": 19795.875,
+ "stations": "Phoenix Harbour,Damask Rose"
+ },
+ {
+ "name": "Cenu Kichs",
+ "pos_x": -15.6875,
+ "pos_y": -63.375,
+ "pos_z": 60.65625,
+ "stations": "Vries Prospect,Fuchs Landing,Cady Beacon"
+ },
+ {
+ "name": "Cenu Ku",
+ "pos_x": 115.34375,
+ "pos_y": -172,
+ "pos_z": 111.71875,
+ "stations": "Clayton Terminal,Opik Horizons,Heinemann Station"
+ },
+ {
+ "name": "Cenufo",
+ "pos_x": -74.6875,
+ "pos_y": -44.65625,
+ "pos_z": 6.6875,
+ "stations": "Crippen Terminal,Faraday Orbital,Winne Gateway,Andrews Terminal,Lethem Barracks,King's Progress"
+ },
+ {
+ "name": "Ceos",
+ "pos_x": -348.65625,
+ "pos_y": 13.875,
+ "pos_z": -339.21875,
+ "stations": "New Dawn Station,Brunel Hub,Babbage Gateway,Sinclair Market"
+ },
+ {
+ "name": "Cephei Sector BA-A d107",
+ "pos_x": -137.25,
+ "pos_y": 28.0625,
+ "pos_z": -64.53125,
+ "stations": "Crown Vision"
+ },
+ {
+ "name": "Cephei Sector BA-A d85",
+ "pos_x": -130.875,
+ "pos_y": 12.34375,
+ "pos_z": -68,
+ "stations": "Favier Prospect,Hasse Relay"
+ },
+ {
+ "name": "Cephei Sector CQ-Y b2",
+ "pos_x": -121.96875,
+ "pos_y": 16.03125,
+ "pos_z": -71.40625,
+ "stations": "Macgregor's Folly"
+ },
+ {
+ "name": "Cephei Sector DL-Y c16",
+ "pos_x": -143.3125,
+ "pos_y": 49.75,
+ "pos_z": -45.3125,
+ "stations": "Zajdel Base,Hamilton's Inheritance"
+ },
+ {
+ "name": "Cephei Sector FG-Y d71",
+ "pos_x": -128.28125,
+ "pos_y": 17.03125,
+ "pos_z": -14.65625,
+ "stations": "Birdseye Survey,Knipling's Folly"
+ },
+ {
+ "name": "Cephei Sector FG-Y d89",
+ "pos_x": -141.9375,
+ "pos_y": 45.75,
+ "pos_z": -10.46875,
+ "stations": "Ostwald's Claim"
+ },
+ {
+ "name": "Cephei Sector FM-V b2-1",
+ "pos_x": -133.25,
+ "pos_y": 61.9375,
+ "pos_z": -38.09375,
+ "stations": "Fuca Terminal,Forrester Installation"
+ },
+ {
+ "name": "Cephei Sector HR-W c1-15",
+ "pos_x": -140.84375,
+ "pos_y": 45.9375,
+ "pos_z": -12.53125,
+ "stations": "Newman Silo"
+ },
+ {
+ "name": "Cephei Sector YZ-Y b4",
+ "pos_x": -119.90625,
+ "pos_y": 58.71875,
+ "pos_z": -75.8125,
+ "stations": "Delany Terminal,Swift's Progress"
+ },
+ {
+ "name": "Cerari",
+ "pos_x": -74.5,
+ "pos_y": -72.125,
+ "pos_z": 128.03125,
+ "stations": "Makarov Settlement,Andreas Survey,Lambert Survey"
+ },
+ {
+ "name": "Ceraunii",
+ "pos_x": 45.40625,
+ "pos_y": -147.59375,
+ "pos_z": -82.25,
+ "stations": "Fokker Port,Reinmuth Station,Fraser Beacon,Kimberlin Vision"
+ },
+ {
+ "name": "Ceri",
+ "pos_x": -45.21875,
+ "pos_y": 81.875,
+ "pos_z": 22.8125,
+ "stations": "Fourier Orbital,Fawcett Terminal,Diesel Camp"
+ },
+ {
+ "name": "Cerktondhs",
+ "pos_x": -5.0625,
+ "pos_y": -23.5625,
+ "pos_z": 59.25,
+ "stations": "Buchli Terminal,Bluford Gateway,Hahn Orbital,Morgan Installation,Leeuwenhoek Hub"
+ },
+ {
+ "name": "Cerni",
+ "pos_x": 145.25,
+ "pos_y": -8,
+ "pos_z": -34.53125,
+ "stations": "Sagan Port,Watson Installation,Vinogradov Dock,Maler Terminal"
+ },
+ {
+ "name": "Cerno",
+ "pos_x": 30.0625,
+ "pos_y": 26.875,
+ "pos_z": -82.9375,
+ "stations": "Stabenow Survey,Kanwar Enterprise,Haisheng Survey,Khan Installation"
+ },
+ {
+ "name": "Cernobog",
+ "pos_x": 77.71875,
+ "pos_y": -140.28125,
+ "pos_z": 47.53125,
+ "stations": "Kirkwood Vision,Henderson Port"
+ },
+ {
+ "name": "Cernunnos",
+ "pos_x": 59.25,
+ "pos_y": -151.6875,
+ "pos_z": 32.5625,
+ "stations": "Bode Hub,Ghez Station,Escobar Installation"
+ },
+ {
+ "name": "Ceroesii",
+ "pos_x": -41.1875,
+ "pos_y": -0.1875,
+ "pos_z": 125.71875,
+ "stations": "Khan Station,Kavandi's Progress,Galton Landing"
+ },
+ {
+ "name": "Ceroklis",
+ "pos_x": -128.53125,
+ "pos_y": -15.875,
+ "pos_z": 18.90625,
+ "stations": "Lasswitz Settlement"
+ },
+ {
+ "name": "Ceronir",
+ "pos_x": 128.8125,
+ "pos_y": -114.53125,
+ "pos_z": 58.03125,
+ "stations": "Mooz Orbital,Juan de la Cierva Orbital,Harvia Holdings,Flagg Market,Nomura Vision,Keeler Terminal,Moore Enterprise,Riccioli Orbital,Check Orbital,Very Landing,Bassford Point"
+ },
+ {
+ "name": "Cerre",
+ "pos_x": -138.875,
+ "pos_y": -60.625,
+ "pos_z": -75.96875,
+ "stations": "Witt Freeport"
+ },
+ {
+ "name": "Cerremayeb",
+ "pos_x": -125.40625,
+ "pos_y": 43.96875,
+ "pos_z": 81.0625,
+ "stations": "Constantine Beacon,Smith Retreat,Pond Keep"
+ },
+ {
+ "name": "Cerrero",
+ "pos_x": -45.375,
+ "pos_y": 80.21875,
+ "pos_z": -125.65625,
+ "stations": "Wundt Hub,Nowak Base"
+ },
+ {
+ "name": "Cerritics",
+ "pos_x": 1,
+ "pos_y": 43.03125,
+ "pos_z": -163.53125,
+ "stations": "Townshend Station,Bloch Penal colony,Brash Vista"
+ },
+ {
+ "name": "Cerrodi",
+ "pos_x": 122.75,
+ "pos_y": -204.5625,
+ "pos_z": -11.21875,
+ "stations": "Courvoisier Port,Flinders' Progress,Crossfield Mines,Yuzhe Enterprise"
+ },
+ {
+ "name": "Cerry",
+ "pos_x": 100.9375,
+ "pos_y": 20.0625,
+ "pos_z": -102.40625,
+ "stations": "Fuglesang Landing"
+ },
+ {
+ "name": "Certo",
+ "pos_x": 22.4375,
+ "pos_y": -26.71875,
+ "pos_z": 85.375,
+ "stations": "Hayden Dock,Lagrange Platform,Joliot-Curie Beacon"
+ },
+ {
+ "name": "Cet",
+ "pos_x": 36.59375,
+ "pos_y": -21.375,
+ "pos_z": -0.78125,
+ "stations": "Yaping Ring,McKay Ring,Prunariu Port,Zoline Beacon"
+ },
+ {
+ "name": "Ceti Sector CL-Y c11",
+ "pos_x": -72.875,
+ "pos_y": -153.0625,
+ "pos_z": -41.0625,
+ "stations": "Barratt Reach"
+ },
+ {
+ "name": "Ceti Sector CL-Y d55",
+ "pos_x": -70.65625,
+ "pos_y": -113.90625,
+ "pos_z": -20.875,
+ "stations": "Verne Settlement,MacLeod Base"
+ },
+ {
+ "name": "Ceti Sector CQ-Y b0",
+ "pos_x": -34.875,
+ "pos_y": -149.5,
+ "pos_z": -71.6875,
+ "stations": "Grego Landing,Goldstein's Progress"
+ },
+ {
+ "name": "Ceti Sector DL-Y c16",
+ "pos_x": -30.53125,
+ "pos_y": -181.53125,
+ "pos_z": -46.1875,
+ "stations": "Shinn Lab,Dyr Mines"
+ },
+ {
+ "name": "Ceti Sector EB-X b1-0",
+ "pos_x": -42.8125,
+ "pos_y": -141.65625,
+ "pos_z": -62.0625,
+ "stations": "Foreman Camp"
+ },
+ {
+ "name": "Ceti Sector EB-X b1-3",
+ "pos_x": -39.21875,
+ "pos_y": -127.09375,
+ "pos_z": -54.65625,
+ "stations": "Siodmak's Inheritance,Hodgson Arsenal"
+ },
+ {
+ "name": "Ceti Sector WO-A c22",
+ "pos_x": -20.9375,
+ "pos_y": -80.4375,
+ "pos_z": -84.5,
+ "stations": "Tudela Vision,Heck's Folly,Lasswitz's Inheritance,Sherrington Horizons"
+ },
+ {
+ "name": "Ceti Sector YJ-A c15",
+ "pos_x": -8.1875,
+ "pos_y": -118.9375,
+ "pos_z": -101.0625,
+ "stations": "Stewart Depot"
+ },
+ {
+ "name": "Ceti Sector ZE-A d74",
+ "pos_x": -35.5,
+ "pos_y": -174.78125,
+ "pos_z": -55.625,
+ "stations": "Dall Installation,Arrhenius Enterprise"
+ },
+ {
+ "name": "Ceti Sector ZE-A d90",
+ "pos_x": -62.34375,
+ "pos_y": -156.78125,
+ "pos_z": -52.59375,
+ "stations": "Beltrami Penal colony"
+ },
+ {
+ "name": "Ceut",
+ "pos_x": 126.1875,
+ "pos_y": -101.125,
+ "pos_z": 67.5625,
+ "stations": "Connes Dock"
+ },
+ {
+ "name": "Ceutrones",
+ "pos_x": -166.5,
+ "pos_y": -23.5,
+ "pos_z": 39.59375,
+ "stations": "Alexander Platform,Gloss Port"
+ },
+ {
+ "name": "Ceuts",
+ "pos_x": 110.21875,
+ "pos_y": -214.125,
+ "pos_z": 108.03125,
+ "stations": "Sato Landing"
+ },
+ {
+ "name": "CF 16001",
+ "pos_x": 30.65625,
+ "pos_y": -8.1875,
+ "pos_z": 85.03125,
+ "stations": "Fremont Prospect,Nusslein-Volhard Station,Swanson Port,Hui Penal colony,Vaucanson Colony,ACS Overwatch"
+ },
+ {
+ "name": "CF 464",
+ "pos_x": 46.90625,
+ "pos_y": -120.15625,
+ "pos_z": 7.1875,
+ "stations": "Bartini Enterprise"
+ },
+ {
+ "name": "CH Aquilae",
+ "pos_x": -68.53125,
+ "pos_y": -51.1875,
+ "pos_z": 80.75,
+ "stations": "Cori Station,Conklin Reach,Akers Ring,Bloomfield Station,Jakes Penal colony"
+ },
+ {
+ "name": "Ch'anjie",
+ "pos_x": 75.96875,
+ "pos_y": 66.09375,
+ "pos_z": 22.75,
+ "stations": "Hopkins Dock,Auld Terminal"
+ },
+ {
+ "name": "Ch'eng",
+ "pos_x": 95.4375,
+ "pos_y": -161.46875,
+ "pos_z": 74.71875,
+ "stations": "Shen Market,Fairey Station"
+ },
+ {
+ "name": "Ch'i",
+ "pos_x": 110.0625,
+ "pos_y": -216.46875,
+ "pos_z": 102.46875,
+ "stations": "Harrison Works"
+ },
+ {
+ "name": "Ch'i Lin",
+ "pos_x": 22.90625,
+ "pos_y": -127.8125,
+ "pos_z": 47.34375,
+ "stations": "Hogg Depot,Lemaitre Dock,Fallows Orbital,Barr Holdings,Challis Vision"
+ },
+ {
+ "name": "Ch'i Ling",
+ "pos_x": 19.75,
+ "pos_y": -200.15625,
+ "pos_z": 19.375,
+ "stations": "McMillan Platform,Petaja Enterprise,Brashear Prospect"
+ },
+ {
+ "name": "Ch'i Lingo",
+ "pos_x": -73.21875,
+ "pos_y": -42.6875,
+ "pos_z": 35.5,
+ "stations": "Linenger Port,Mastracchio Prospect"
+ },
+ {
+ "name": "Ch'ian",
+ "pos_x": 68.125,
+ "pos_y": 112.46875,
+ "pos_z": -78.5,
+ "stations": "Conway Orbital,Wagner Dock,Nagata Horizons,Elvstrom Beacon"
+ },
+ {
+ "name": "Ch'ian Hsia",
+ "pos_x": 136.1875,
+ "pos_y": -178.1875,
+ "pos_z": -2.3125,
+ "stations": "Wegener Beacon,Bernoulli Plant,Vuia Landing"
+ },
+ {
+ "name": "Ch'iang Fei",
+ "pos_x": 84.28125,
+ "pos_y": -4.0625,
+ "pos_z": 71.8125,
+ "stations": "Fisher Hub,Leopold Settlement,Cortes Base,Ejeta Enterprise,Cleave Vision"
+ },
+ {
+ "name": "Ch'in Shou",
+ "pos_x": 99.5,
+ "pos_y": -33.5,
+ "pos_z": -40.34375,
+ "stations": "Emshwiller Installation,Alas Dock,Kavandi Enterprise,Blenkinsop Terminal,Gordon Hub,Sellers Reach"
+ },
+ {
+ "name": "Ch'in Yang",
+ "pos_x": 67.4375,
+ "pos_y": -95.9375,
+ "pos_z": -2.1875,
+ "stations": "Dumont Terminal"
+ },
+ {
+ "name": "Ch'ing",
+ "pos_x": 48.78125,
+ "pos_y": -48.625,
+ "pos_z": -119.625,
+ "stations": "Hiroyuki Relay,Drake Landing"
+ },
+ {
+ "name": "Ch'ingsonde",
+ "pos_x": -114.03125,
+ "pos_y": 21.59375,
+ "pos_z": 55.03125,
+ "stations": "Bixby Depot,McArthur City,Kafka Enterprise,Schrodinger Orbital,Solovyov Gateway"
+ },
+ {
+ "name": "Ch'ol",
+ "pos_x": -88.4375,
+ "pos_y": 61.375,
+ "pos_z": 160.15625,
+ "stations": "Cook Dock,Gessi Landing,Quaglia Colony,Wait Colburn Base"
+ },
+ {
+ "name": "Ch'ortae",
+ "pos_x": -98.84375,
+ "pos_y": -59,
+ "pos_z": 97.59375,
+ "stations": "Fast Outpost"
+ },
+ {
+ "name": "Ch'ortamaye",
+ "pos_x": -107.40625,
+ "pos_y": 11.875,
+ "pos_z": -149.59375,
+ "stations": "Keyes Platform,Wnuk-Lipinski Settlement,Linaweaver Point"
+ },
+ {
+ "name": "Ch'u Kiang Wang",
+ "pos_x": 150.625,
+ "pos_y": -126.34375,
+ "pos_z": 112.125,
+ "stations": "Paczynski Terminal,Arrhenius Dock,Wilhelm von Struve Keep"
+ },
+ {
+ "name": "Ch'u Ti",
+ "pos_x": -101.5625,
+ "pos_y": -83.875,
+ "pos_z": 37.96875,
+ "stations": "Fincke Hub,Snyder Landing"
+ },
+ {
+ "name": "Ch'uanga",
+ "pos_x": -85.0625,
+ "pos_y": -20.21875,
+ "pos_z": 33.1875,
+ "stations": "Jordan Dock,Pelliot Settlement,Barr City,Pullman Terminal"
+ },
+ {
+ "name": "Ch'unchuan",
+ "pos_x": 56.0625,
+ "pos_y": -98.3125,
+ "pos_z": 102.34375,
+ "stations": "Somerville Dock"
+ },
+ {
+ "name": "Ch'unga",
+ "pos_x": 140.03125,
+ "pos_y": -156.46875,
+ "pos_z": 10.15625,
+ "stations": "Tyson City,Zeppelin Dock,Fox Terminal"
+ },
+ {
+ "name": "Chaac",
+ "pos_x": 0.4375,
+ "pos_y": 63.21875,
+ "pos_z": -9.5,
+ "stations": "Lem Dock,Gerlache Installation,Gernsback Relay"
+ },
+ {
+ "name": "Chaaratisa",
+ "pos_x": -33.90625,
+ "pos_y": -122.875,
+ "pos_z": 7.3125,
+ "stations": "Turner Station,Rich Terminal,Frazetta Gateway,Peebles City,Rocklynne Horizons,Aitken Gateway,Bailly City,Grimwood Relay,Utkin Port,Lockyer Relay,Potagos Installation"
+ },
+ {
+ "name": "Chac",
+ "pos_x": 66.65625,
+ "pos_y": 32.09375,
+ "pos_z": -52.71875,
+ "stations": "Grassmann Orbital,Klein Dock"
+ },
+ {
+ "name": "Chac Cimih",
+ "pos_x": 68.71875,
+ "pos_y": 52.4375,
+ "pos_z": 94.09375,
+ "stations": "Busch Ring,Blackman Dock,Abernathy Ring,Oxley Terminal"
+ },
+ {
+ "name": "Chac Cimil",
+ "pos_x": 60.875,
+ "pos_y": 34.875,
+ "pos_z": 86.3125,
+ "stations": "Appel Depot"
+ },
+ {
+ "name": "Chacahua",
+ "pos_x": -24.34375,
+ "pos_y": -92.09375,
+ "pos_z": 113.40625,
+ "stations": "Schlesinger Terminal,Heinkel Landing,Check Terminal"
+ },
+ {
+ "name": "Chachapoyas",
+ "pos_x": 52.25,
+ "pos_y": -174.21875,
+ "pos_z": 95.40625,
+ "stations": "De Hub,Citi Settlement"
+ },
+ {
+ "name": "Chacobo",
+ "pos_x": 121.65625,
+ "pos_y": -159.53125,
+ "pos_z": -23.4375,
+ "stations": "Matthaus Olbers Terminal,Rubin Terminal,Cellarius Observatory"
+ },
+ {
+ "name": "Chacobog",
+ "pos_x": -161.15625,
+ "pos_y": 27.375,
+ "pos_z": -72.75,
+ "stations": "Trinh Enterprise,Finney Holdings"
+ },
+ {
+ "name": "Chacoc",
+ "pos_x": 7.84375,
+ "pos_y": 146.34375,
+ "pos_z": 22.59375,
+ "stations": "Hughes Landing,Pratchett Legacy"
+ },
+ {
+ "name": "Chacocels",
+ "pos_x": 99.90625,
+ "pos_y": -143.6875,
+ "pos_z": -51.15625,
+ "stations": "Emshwiller Station,Gotz Port"
+ },
+ {
+ "name": "Chacocha",
+ "pos_x": 26.9375,
+ "pos_y": -81,
+ "pos_z": 61.875,
+ "stations": "O'Neill Settlement,Tarski Prospect,Purbach Holdings"
+ },
+ {
+ "name": "Chacorii",
+ "pos_x": 124.25,
+ "pos_y": -198.375,
+ "pos_z": -59.6875,
+ "stations": "Muramatsu Mine,Mason Depot"
+ },
+ {
+ "name": "Chaga",
+ "pos_x": 62.6875,
+ "pos_y": 68.375,
+ "pos_z": -56.28125,
+ "stations": "Biggle City,Hayden Gateway,Bierce Port"
+ },
+ {
+ "name": "Chagin",
+ "pos_x": 81.625,
+ "pos_y": 20.65625,
+ "pos_z": -43.125,
+ "stations": "Macquorn Rankine Works,Tyson Plant,Al-Khalili Relay"
+ },
+ {
+ "name": "Chagur",
+ "pos_x": -0.90625,
+ "pos_y": -204.8125,
+ "pos_z": 119.09375,
+ "stations": "Kidinnu Mines,Arend Vision,Mrkos Town"
+ },
+ {
+ "name": "Chaimal",
+ "pos_x": -72.40625,
+ "pos_y": -19.03125,
+ "pos_z": -117.8125,
+ "stations": "Strekalov Landing,Shepard Ring,J. G. Ballard Hub,Roddenberry Enterprise,Fermi Settlement"
+ },
+ {
+ "name": "Chaiman",
+ "pos_x": 50.125,
+ "pos_y": -194.25,
+ "pos_z": 63.53125,
+ "stations": "Hulse Port"
+ },
+ {
+ "name": "Chainandra",
+ "pos_x": 156.9375,
+ "pos_y": -164.09375,
+ "pos_z": 9.5625,
+ "stations": "Whittle Reformatory"
+ },
+ {
+ "name": "Chairhuhyus",
+ "pos_x": -78.65625,
+ "pos_y": -28.21875,
+ "pos_z": 28.78125,
+ "stations": "Creamer Terminal"
+ },
+ {
+ "name": "Chairipatis",
+ "pos_x": 119.25,
+ "pos_y": -163,
+ "pos_z": 28.96875,
+ "stations": "Kopal Terminal,Titius Base"
+ },
+ {
+ "name": "Chaitany",
+ "pos_x": 44.53125,
+ "pos_y": -235.09375,
+ "pos_z": 86.75,
+ "stations": "Schmidt Dock,Digges Vision"
+ },
+ {
+ "name": "Chaiya",
+ "pos_x": -87.71875,
+ "pos_y": 42.8125,
+ "pos_z": 11.46875,
+ "stations": "Redi Port,Vetulani Terminal,Gregory Hub,Reamy Depot"
+ },
+ {
+ "name": "Chaiyan",
+ "pos_x": 165.40625,
+ "pos_y": -122.40625,
+ "pos_z": 0.65625,
+ "stations": "Honda Station,Knorre Landing,Kalam Enterprise"
+ },
+ {
+ "name": "Chak",
+ "pos_x": 82.1875,
+ "pos_y": -216.90625,
+ "pos_z": 113.25,
+ "stations": "Jael Base"
+ },
+ {
+ "name": "Chakaia",
+ "pos_x": -154.96875,
+ "pos_y": 68.375,
+ "pos_z": 28.375,
+ "stations": "Margulies Dock,Smith Silo"
+ },
+ {
+ "name": "Chakho",
+ "pos_x": 44.75,
+ "pos_y": -191.71875,
+ "pos_z": 32.34375,
+ "stations": "Ayers Stop,Cellarius Plant"
+ },
+ {
+ "name": "Chakma",
+ "pos_x": -150.0625,
+ "pos_y": -40.78125,
+ "pos_z": -23.1875,
+ "stations": "Dunbar Holdings"
+ },
+ {
+ "name": "Chakmo",
+ "pos_x": -97.5,
+ "pos_y": 9.09375,
+ "pos_z": 59.03125,
+ "stations": "Kornbluth Hangar,Scithers Plant"
+ },
+ {
+ "name": "Chakpa",
+ "pos_x": 30.1875,
+ "pos_y": 145.03125,
+ "pos_z": 58.03125,
+ "stations": "Dunbar Survey,Williams Dock,Chargaff Installation"
+ },
+ {
+ "name": "Chakul",
+ "pos_x": -70.1875,
+ "pos_y": -114.125,
+ "pos_z": 40.8125,
+ "stations": "Babbage Settlement,Payton Platform"
+ },
+ {
+ "name": "Chakyum",
+ "pos_x": -131.75,
+ "pos_y": -14.15625,
+ "pos_z": -22.25,
+ "stations": "Lyell Port,Boyle Port,Brooks Works,Sellers Gateway,Bentham Prospect"
+ },
+ {
+ "name": "Chalbandia",
+ "pos_x": 105.34375,
+ "pos_y": -115.96875,
+ "pos_z": 8.625,
+ "stations": "Meuron Station,Hansen Station"
+ },
+ {
+ "name": "Chalbici",
+ "pos_x": -107.96875,
+ "pos_y": 23.84375,
+ "pos_z": 102.875,
+ "stations": "Hale Horizons,Meade Platform"
+ },
+ {
+ "name": "Chaman",
+ "pos_x": 138.09375,
+ "pos_y": 31.25,
+ "pos_z": 14.46875,
+ "stations": "Lee City,Converse Vision,Isherwood Hub"
+ },
+ {
+ "name": "Chamas",
+ "pos_x": 79.28125,
+ "pos_y": 40.25,
+ "pos_z": 153.125,
+ "stations": "Denton Dock,Harrison Dock"
+ },
+ {
+ "name": "Chamath",
+ "pos_x": 61.25,
+ "pos_y": 43.8125,
+ "pos_z": 135.875,
+ "stations": "Kennicott Hub,Altshuller Orbital,Grothendieck Orbital,Kelleam Installation"
+ },
+ {
+ "name": "Chambo",
+ "pos_x": 34.3125,
+ "pos_y": -24.875,
+ "pos_z": 24.28125,
+ "stations": "Deluc Settlement,Deb Settlement,Robinson Point"
+ },
+ {
+ "name": "Chamo",
+ "pos_x": -26.46875,
+ "pos_y": -124.15625,
+ "pos_z": -46.46875,
+ "stations": "Martiniere Port,Fan Port,Frost Terminal,Heinkel City,Merril Beacon,Nagata Station"
+ },
+ {
+ "name": "Chamonjurui",
+ "pos_x": 2.03125,
+ "pos_y": -209.65625,
+ "pos_z": 117.40625,
+ "stations": "Evans Terminal,Muramatsu Colony"
+ },
+ {
+ "name": "Chamuluma",
+ "pos_x": 105.90625,
+ "pos_y": -90.46875,
+ "pos_z": 19.90625,
+ "stations": "Whitney Orbital,Bisnovatyi-Kogan Landing,Wilhelm Vision"
+ },
+ {
+ "name": "Chamunda",
+ "pos_x": -47.21875,
+ "pos_y": -6.78125,
+ "pos_z": 62.125,
+ "stations": "Gidzenko Ring"
+ },
+ {
+ "name": "Chan Mina",
+ "pos_x": -3.65625,
+ "pos_y": 19.6875,
+ "pos_z": -101.5,
+ "stations": "Vishweswarayya Port"
+ },
+ {
+ "name": "Chana",
+ "pos_x": 103.75,
+ "pos_y": -43.90625,
+ "pos_z": -40.4375,
+ "stations": "Swigert Hub,Kooi Hub,Moran Arsenal,Saberhagen Base,Wedge Orbital,Ross Station,Schweickart Orbital,McArthur Terminal,al-Khowarizmi Lab,Trevithick Dock"
+ },
+ {
+ "name": "Chanab",
+ "pos_x": 23,
+ "pos_y": -64.5,
+ "pos_z": 152.34375,
+ "stations": "Alexandria Dock"
+ },
+ {
+ "name": "Chanarsi",
+ "pos_x": 57.71875,
+ "pos_y": -140.625,
+ "pos_z": 104.9375,
+ "stations": "Giancola Prospect,Michell Orbital,Smyth Orbital,Houten-Groeneveld Landing"
+ },
+ {
+ "name": "Chanasar",
+ "pos_x": 135.78125,
+ "pos_y": -78,
+ "pos_z": -40.40625,
+ "stations": "Meuron Mines"
+ },
+ {
+ "name": "Chande",
+ "pos_x": -78.28125,
+ "pos_y": 119,
+ "pos_z": 33.21875,
+ "stations": "Heng Port,Anning Dock,Kregel Colony,McClintock's Inheritance,Blair Silo"
+ },
+ {
+ "name": "Chandh",
+ "pos_x": 97,
+ "pos_y": -3.90625,
+ "pos_z": 23.5625,
+ "stations": "Lavoisier Settlement,Wang Works"
+ },
+ {
+ "name": "Chandra",
+ "pos_x": 8.84375,
+ "pos_y": 47.34375,
+ "pos_z": -58.59375,
+ "stations": "Schmitt Terminal,Laplace Station,Shull Station,Panshin Installation,Blalock Installation"
+ },
+ {
+ "name": "Chang",
+ "pos_x": -35.3125,
+ "pos_y": -154.90625,
+ "pos_z": -0.84375,
+ "stations": "Darboux's Folly,Pritchard Landing,Roemer Hub"
+ },
+ {
+ "name": "Chang Hsien",
+ "pos_x": 20.78125,
+ "pos_y": -105.84375,
+ "pos_z": 105.875,
+ "stations": "Sturgeon Depot,Bigourdan Installation,Lagerkvist Prospect,Thiele Hub"
+ },
+ {
+ "name": "Chang Kuo Lao",
+ "pos_x": -77.34375,
+ "pos_y": -15.1875,
+ "pos_z": 116.5625,
+ "stations": "Moon Dock"
+ },
+ {
+ "name": "Chang O",
+ "pos_x": 2.625,
+ "pos_y": 27.5625,
+ "pos_z": -154.375,
+ "stations": "Hirase Colony,Siemens Base"
+ },
+ {
+ "name": "Chang Shor",
+ "pos_x": -85.96875,
+ "pos_y": 15,
+ "pos_z": 33.75,
+ "stations": "Baydukov Platform,Menezes Installation,Stanley Plant"
+ },
+ {
+ "name": "Chang Yeh",
+ "pos_x": 25.6875,
+ "pos_y": -4.8125,
+ "pos_z": -50.53125,
+ "stations": "Nicollet City,Blaschke Vision,Monge Relay,Bear Silo"
+ },
+ {
+ "name": "Changai",
+ "pos_x": -32.4375,
+ "pos_y": 27.78125,
+ "pos_z": 136.625,
+ "stations": "Narita Dock,Holden Station,Kippax Port,Kerr Dock,Caselberg Base,Roberts Refinery,Mitra Dock"
+ },
+ {
+ "name": "Changbatzil",
+ "pos_x": 45.21875,
+ "pos_y": -108.625,
+ "pos_z": -127.5625,
+ "stations": "Sawyer Platform,Shalatula's Claim,Heck Base"
+ },
+ {
+ "name": "Changda",
+ "pos_x": 21.78125,
+ "pos_y": 63.6875,
+ "pos_z": 79.15625,
+ "stations": "Ali Survey,Bondar Mines,Kimbrough Survey,Steele Port"
+ },
+ {
+ "name": "Changgu",
+ "pos_x": -33.125,
+ "pos_y": -35.3125,
+ "pos_z": 35.1875,
+ "stations": "Thornton Survey,Mendeleev Colony,Arber Reach,Braun Landing"
+ },
+ {
+ "name": "Changkhulym",
+ "pos_x": 141.375,
+ "pos_y": -28.875,
+ "pos_z": 44.125,
+ "stations": "Miller Dock"
+ },
+ {
+ "name": "Changthini",
+ "pos_x": -4.625,
+ "pos_y": -9.15625,
+ "pos_z": 63.34375,
+ "stations": "Walker Platform,Shkaplerov Hangar,Popper's Folly"
+ },
+ {
+ "name": "Changur",
+ "pos_x": 37.84375,
+ "pos_y": -60.25,
+ "pos_z": 111.34375,
+ "stations": "Tyson Orbital,Houten-Groeneveld Bastion"
+ },
+ {
+ "name": "Changwuti",
+ "pos_x": 115.90625,
+ "pos_y": -157.8125,
+ "pos_z": 100.65625,
+ "stations": "Valigursky Works,Dawson Mine"
+ },
+ {
+ "name": "Chanii",
+ "pos_x": 55.875,
+ "pos_y": 71.90625,
+ "pos_z": 127.875,
+ "stations": "Lundwall Hub,Green Colony,Paulmier de Gonneville Settlement"
+ },
+ {
+ "name": "Chankates",
+ "pos_x": 99.75,
+ "pos_y": -24.5,
+ "pos_z": 122.8125,
+ "stations": "Alcala Arsenal,Piccard Settlement"
+ },
+ {
+ "name": "Chankopa",
+ "pos_x": -164.0625,
+ "pos_y": 6.6875,
+ "pos_z": -12.5625,
+ "stations": "Henderson Depot,Herndon Stop"
+ },
+ {
+ "name": "Chankun",
+ "pos_x": -19.59375,
+ "pos_y": -76.125,
+ "pos_z": -25.625,
+ "stations": "Melnick Market,Barnes Barracks"
+ },
+ {
+ "name": "Chans",
+ "pos_x": -53.3125,
+ "pos_y": 53.6875,
+ "pos_z": 90.6875,
+ "stations": "Zebrowski Legacy"
+ },
+ {
+ "name": "Chanses",
+ "pos_x": -21.875,
+ "pos_y": -109.375,
+ "pos_z": 72,
+ "stations": "Rutherford Ring"
+ },
+ {
+ "name": "Chanyaya",
+ "pos_x": 132.625,
+ "pos_y": -161.34375,
+ "pos_z": -85.375,
+ "stations": "Barlowe Terminal,Heiles Port,Young Park,Francisco de Eliza Vision"
+ },
+ {
+ "name": "Chanyinyak",
+ "pos_x": 135.71875,
+ "pos_y": -99.84375,
+ "pos_z": -41.75,
+ "stations": "Marsden Dock"
+ },
+ {
+ "name": "Chao Gu",
+ "pos_x": -54.875,
+ "pos_y": -46.21875,
+ "pos_z": 139.21875,
+ "stations": "Potagos Horizons,Zettel Bastion"
+ },
+ {
+ "name": "Chao T'ien",
+ "pos_x": 25,
+ "pos_y": -179.53125,
+ "pos_z": 166.28125,
+ "stations": "Faber Colony"
+ },
+ {
+ "name": "Chao Wu",
+ "pos_x": -2.5,
+ "pos_y": -114.28125,
+ "pos_z": -13.9375,
+ "stations": "Tanaka Port,Heyerdahl Vision,Harrington Dock,Rosseland Settlement,Belyavsky Gateway"
+ },
+ {
+ "name": "Chaona",
+ "pos_x": 66.9375,
+ "pos_y": 64.96875,
+ "pos_z": -39.5,
+ "stations": "Weil Enterprise,Lee Dock,Zamyatin Dock,Bouch Horizons,Derleth Base"
+ },
+ {
+ "name": "Chapo",
+ "pos_x": -93.28125,
+ "pos_y": 62.96875,
+ "pos_z": -32.0625,
+ "stations": "Fincke Platform,Jones Beacon,Ali Port"
+ },
+ {
+ "name": "Chapoyo",
+ "pos_x": -100.375,
+ "pos_y": 34.46875,
+ "pos_z": -40.09375,
+ "stations": "Richards Settlement"
+ },
+ {
+ "name": "Chapsugai",
+ "pos_x": 29.03125,
+ "pos_y": -100.96875,
+ "pos_z": -43,
+ "stations": "Zhigang Platform,Mawson Base,Shaw Base"
+ },
+ {
+ "name": "Chapsugaibo",
+ "pos_x": 42.5625,
+ "pos_y": -80.78125,
+ "pos_z": 10.0625,
+ "stations": "Schlesinger City,Borrego Port"
+ },
+ {
+ "name": "Chapsugs",
+ "pos_x": 97.84375,
+ "pos_y": -1.8125,
+ "pos_z": -123,
+ "stations": "Jones Depot"
+ },
+ {
+ "name": "Charang",
+ "pos_x": -82.96875,
+ "pos_y": -84.21875,
+ "pos_z": -119.53125,
+ "stations": "Bellamy Terminal,Jordan Outpost,Brooks Vision"
+ },
+ {
+ "name": "Charao",
+ "pos_x": 72.78125,
+ "pos_y": 2.84375,
+ "pos_z": -144.0625,
+ "stations": "Heyerdahl Settlement"
+ },
+ {
+ "name": "Charenner",
+ "pos_x": 52.875,
+ "pos_y": 79.3125,
+ "pos_z": 58.21875,
+ "stations": "Kennan Orbital,King Arsenal"
+ },
+ {
+ "name": "Charonium",
+ "pos_x": 39.8125,
+ "pos_y": -7.59375,
+ "pos_z": -25.3125,
+ "stations": "Galouye Landing,Pullman Base,Eskridge Terminal"
+ },
+ {
+ "name": "Charunder",
+ "pos_x": 38.53125,
+ "pos_y": -10.125,
+ "pos_z": 11.625,
+ "stations": "Wilson Penal colony,Chomsky Terminal,Mattingly City,Treshchov Market,Karl Diesel Port,Onufriyenko City,Whitworth Station,Leestma Station,Behnken Orbital,Lavoisier Enterprise,Janes Reach,Agassiz Plant,Laliberte Laboratory,McClintock Orbital,Stephens Prospect"
+ },
+ {
+ "name": "Chati",
+ "pos_x": 164.375,
+ "pos_y": -83.96875,
+ "pos_z": 55.40625,
+ "stations": "Woodroffe Gateway,van der Riet Woolley Ring,Silva Horizons,Maskelyne Dock,Kulin Installation"
+ },
+ {
+ "name": "Chatkwa",
+ "pos_x": -126.15625,
+ "pos_y": 60.84375,
+ "pos_z": 93.875,
+ "stations": "Fullerton Outpost,Santos Vision,Wheelock Platform"
+ },
+ {
+ "name": "Chau Yu",
+ "pos_x": 90.96875,
+ "pos_y": -192.53125,
+ "pos_z": -3.5625,
+ "stations": "Akiyama Ring,Miller Orbital,Parkinson Port,Whitney Installation,Herbig Arsenal"
+ },
+ {
+ "name": "Chaualkyrja",
+ "pos_x": 71.21875,
+ "pos_y": 150.71875,
+ "pos_z": 16.8125,
+ "stations": "Thomson Landing,Conway City"
+ },
+ {
+ "name": "Chaxiraxi",
+ "pos_x": -9.84375,
+ "pos_y": 66.53125,
+ "pos_z": 5.75,
+ "stations": "Metcalf Orbital,de Sousa Landing,Gamow Orbital,Liska Dock,Rondon Beacon"
+ },
+ {
+ "name": "Chayahun Di",
+ "pos_x": -33.71875,
+ "pos_y": -168.1875,
+ "pos_z": 19.40625,
+ "stations": "Nearchus Landing,Rodrigues Terminal,Kimura Hub,Nilson Orbital,Struve Hub"
+ },
+ {
+ "name": "Chayansi",
+ "pos_x": -44.1875,
+ "pos_y": -69.71875,
+ "pos_z": 60.09375,
+ "stations": "Bernoulli Hangar"
+ },
+ {
+ "name": "Chayuse",
+ "pos_x": -67.15625,
+ "pos_y": 104.78125,
+ "pos_z": -9.875,
+ "stations": "Valdes Enterprise,Asire Landing"
+ },
+ {
+ "name": "Chebaei",
+ "pos_x": 21.375,
+ "pos_y": -205.71875,
+ "pos_z": -22.53125,
+ "stations": "Bulychev Beacon,Milne Enterprise,Hughes Vision"
+ },
+ {
+ "name": "Chebay",
+ "pos_x": -42.5,
+ "pos_y": -141.40625,
+ "pos_z": 131.46875,
+ "stations": "Kraft Base,Laing Enterprise,Davis Enterprise"
+ },
+ {
+ "name": "Checha",
+ "pos_x": 18.3125,
+ "pos_y": -67.25,
+ "pos_z": 146.21875,
+ "stations": "Gehry Gateway,Riebe Enterprise,Auld Silo,Digges Terminal,Caidin Base"
+ },
+ {
+ "name": "Chechehet",
+ "pos_x": -106.5625,
+ "pos_y": 24.625,
+ "pos_z": -27.90625,
+ "stations": "Desargues Works,Hand Base"
+ },
+ {
+ "name": "Chedians",
+ "pos_x": 56.25,
+ "pos_y": -181.84375,
+ "pos_z": -3.6875,
+ "stations": "Ikeya Port,Shavyrin Prospect,Mikoyan Horizons"
+ },
+ {
+ "name": "Chediq",
+ "pos_x": 112.0625,
+ "pos_y": 33.90625,
+ "pos_z": 8.84375,
+ "stations": "Kessel Platform,Brooks Survey,Ziemianski Survey"
+ },
+ {
+ "name": "Chedita",
+ "pos_x": -27.5,
+ "pos_y": -130.625,
+ "pos_z": 28.3125,
+ "stations": "Fehrenbach Terminal,Galle Station,Arai Hub,Frechet Beacon"
+ },
+ {
+ "name": "Cheditjala",
+ "pos_x": 111.625,
+ "pos_y": -56,
+ "pos_z": -126.09375,
+ "stations": "Coney Prospect,Low Relay"
+ },
+ {
+ "name": "Chehe",
+ "pos_x": 25.90625,
+ "pos_y": -91.875,
+ "pos_z": 38.71875,
+ "stations": "Hansen Vision"
+ },
+ {
+ "name": "Chehogg",
+ "pos_x": 144.125,
+ "pos_y": 22.34375,
+ "pos_z": 20.0625,
+ "stations": "Detmer Depot,Polansky Settlement"
+ },
+ {
+ "name": "Chehoong Po",
+ "pos_x": 74.46875,
+ "pos_y": -82.34375,
+ "pos_z": 0.5,
+ "stations": "van de Sande Bakhuyzen Settlement,Fowler Vision"
+ },
+ {
+ "name": "Chehoonggu",
+ "pos_x": 65.65625,
+ "pos_y": 65.4375,
+ "pos_z": 30.5625,
+ "stations": "Rashid Station,Bresnik Port"
+ },
+ {
+ "name": "Chel",
+ "pos_x": -33.34375,
+ "pos_y": -74.53125,
+ "pos_z": -64.375,
+ "stations": "Quimper Landing,Grassmann's Folly,Ford Platform"
+ },
+ {
+ "name": "Chelgit",
+ "pos_x": 6.21875,
+ "pos_y": -33.5,
+ "pos_z": 56.21875,
+ "stations": "McBride Base,Humboldt Hub,Elion City,Grabe Orbital,Nicholson's Folly"
+ },
+ {
+ "name": "Chelidones",
+ "pos_x": 59.15625,
+ "pos_y": -24.75,
+ "pos_z": 40.5,
+ "stations": "Steakley Settlement,Krupkat Landing,Zettel Port"
+ },
+ {
+ "name": "Chelini",
+ "pos_x": -86.34375,
+ "pos_y": 133.875,
+ "pos_z": -13.90625,
+ "stations": "Atwood Landing"
+ },
+ {
+ "name": "Chelka",
+ "pos_x": -114.375,
+ "pos_y": 61.90625,
+ "pos_z": -77.25,
+ "stations": "Farrer Settlement"
+ },
+ {
+ "name": "Chelmen",
+ "pos_x": -65.96875,
+ "pos_y": 47.78125,
+ "pos_z": -56.875,
+ "stations": "Emshwiller Hub,Miletus Terminal,Serebrov Observatory,Carpini Horizons,Toll Horizons"
+ },
+ {
+ "name": "Chelmenta",
+ "pos_x": -1.71875,
+ "pos_y": 52.6875,
+ "pos_z": 143.71875,
+ "stations": "Korzun Enterprise,Oswald Station"
+ },
+ {
+ "name": "Chelmentes",
+ "pos_x": -97.71875,
+ "pos_y": 131.0625,
+ "pos_z": 97.6875,
+ "stations": "Fermat Vision,Palmer Terminal,Lichtenberg's Progress"
+ },
+ {
+ "name": "Chelmir",
+ "pos_x": 170.0625,
+ "pos_y": 26.125,
+ "pos_z": -15.5625,
+ "stations": "Lewis Landing,Popov's Claim"
+ },
+ {
+ "name": "Chem",
+ "pos_x": 72.4375,
+ "pos_y": -24.25,
+ "pos_z": 71.75,
+ "stations": "Kizim Port,Nespoli Barracks,Roggeveen Camp"
+ },
+ {
+ "name": "Chemaku",
+ "pos_x": -77.5625,
+ "pos_y": 6.5625,
+ "pos_z": -10.53125,
+ "stations": "Crampton Port,Kaku Orbital,Lawson Station,Ewald City,Ferguson City,Nelson Legacy,Watts Depot,Heaviside Survey"
+ },
+ {
+ "name": "Chemaluk",
+ "pos_x": 66.8125,
+ "pos_y": 61.625,
+ "pos_z": 64.5625,
+ "stations": "Virts Dock,Wollheim's Inheritance,Pogue Station,Tereshkova Enterprise,Gantt's Folly"
+ },
+ {
+ "name": "Chematja",
+ "pos_x": -136.40625,
+ "pos_y": 60.53125,
+ "pos_z": -84.75,
+ "stations": "Swift Mines,Legendre Terminal"
+ },
+ {
+ "name": "Chemaya",
+ "pos_x": 36.5625,
+ "pos_y": -50.8125,
+ "pos_z": -154,
+ "stations": "Baffin City,Drake Orbital,Herbert's Inheritance"
+ },
+ {
+ "name": "Chemez",
+ "pos_x": 139.78125,
+ "pos_y": -191.53125,
+ "pos_z": -18.5625,
+ "stations": "Cayley Dock,Maler Reach"
+ },
+ {
+ "name": "Chen Shapa",
+ "pos_x": -19.53125,
+ "pos_y": -181.5,
+ "pos_z": -50.0625,
+ "stations": "Maskelyne Platform,Kirshner's Inheritance"
+ },
+ {
+ "name": "Chen Zaihe",
+ "pos_x": -36.96875,
+ "pos_y": 117.15625,
+ "pos_z": 52.25,
+ "stations": "Stableford Platform,Rosenberg Settlement"
+ },
+ {
+ "name": "Chenchu",
+ "pos_x": -69.59375,
+ "pos_y": 98.375,
+ "pos_z": 43.3125,
+ "stations": "H. G. Wells Beacon,Hinz Prospect"
+ },
+ {
+ "name": "Cheneredawa",
+ "pos_x": -86.25,
+ "pos_y": -66.15625,
+ "pos_z": -125.875,
+ "stations": "Tarentum City,Koch Landing,Phillips Palace"
+ },
+ {
+ "name": "Cheneti",
+ "pos_x": 26.9375,
+ "pos_y": -77.625,
+ "pos_z": 66.78125,
+ "stations": "Speke Survey,South Port,Wirtanen Port,Cyrrhus Port,Kirk Market,Bolton Hub,Grushin Port,Hammel Lab,Kimberlin Vision,Thurston Landing,Longomontanus Orbital"
+ },
+ {
+ "name": "Cheng",
+ "pos_x": 127.90625,
+ "pos_y": -60.8125,
+ "pos_z": -58.75,
+ "stations": "Matthaus Olbers Platform,Shen Orbital,Whitford Beacon"
+ },
+ {
+ "name": "Cheng Den",
+ "pos_x": -42.875,
+ "pos_y": -69.15625,
+ "pos_z": 25.4375,
+ "stations": "Panshin Station,Hutchinson Station,Auld Market,Chilton Beacon,Gallun Vista,Shiras Relay,Walter Camp"
+ },
+ {
+ "name": "Chenghan",
+ "pos_x": 40.46875,
+ "pos_y": -134.5625,
+ "pos_z": 120.625,
+ "stations": "Hoffleit Port,Zacuto Penal colony"
+ },
+ {
+ "name": "Chengurr",
+ "pos_x": -57.5625,
+ "pos_y": -109.28125,
+ "pos_z": -69.5,
+ "stations": "Hilmers Terminal,Dana City,Crown Point,Vizcaino Relay"
+ },
+ {
+ "name": "Chenses",
+ "pos_x": 17.375,
+ "pos_y": -36.25,
+ "pos_z": -101.3125,
+ "stations": "Lyakhov Dock,Haignere Installation,Bernard Penal colony"
+ },
+ {
+ "name": "Chentiiq",
+ "pos_x": -162.84375,
+ "pos_y": -15.03125,
+ "pos_z": 75.5625,
+ "stations": "Desargues Survey"
+ },
+ {
+ "name": "Chentrima",
+ "pos_x": -112.15625,
+ "pos_y": 70.65625,
+ "pos_z": -94.15625,
+ "stations": "Klimuk Vision,Gagarin Platform,Krupkat Vision"
+ },
+ {
+ "name": "Cheper",
+ "pos_x": 172.84375,
+ "pos_y": -9.53125,
+ "pos_z": 23.3125,
+ "stations": "Bugrov Terminal,Swanwick Terminal"
+ },
+ {
+ "name": "Cherang",
+ "pos_x": -43.84375,
+ "pos_y": -146.5,
+ "pos_z": -35.125,
+ "stations": "Henry Camp,Swift Prospect,Stjepan Seljan Base"
+ },
+ {
+ "name": "Cherbones",
+ "pos_x": 5.1875,
+ "pos_y": 84.53125,
+ "pos_z": -16.75,
+ "stations": "Stasheff Depot,Hume Orbital,Chalker Landing"
+ },
+ {
+ "name": "Chere",
+ "pos_x": 144.21875,
+ "pos_y": -41.34375,
+ "pos_z": 117.375,
+ "stations": "Check Port,Flammarion Vision,Samos Vision,Adams City,Henson's Folly"
+ },
+ {
+ "name": "Cherets",
+ "pos_x": -65.9375,
+ "pos_y": 35.53125,
+ "pos_z": -68.71875,
+ "stations": "Goeppert-Mayer City,Buckey Hub,Virtanen Hub,Koch Hub,Hermaszewski Station,Hieb Dock,Baker Bastion,Denton Prospect,Bond Beacon,Borlaug Gateway"
+ },
+ {
+ "name": "Chernobo",
+ "pos_x": -96.875,
+ "pos_y": 59.8125,
+ "pos_z": -82.625,
+ "stations": "Ellis Settlement,Laveykin Platform"
+ },
+ {
+ "name": "Chersem",
+ "pos_x": 46.53125,
+ "pos_y": 17.125,
+ "pos_z": 136.71875,
+ "stations": "Bruce Colony,Hugh Stop,Malchiodi Prospect"
+ },
+ {
+ "name": "Chert",
+ "pos_x": 115.90625,
+ "pos_y": -75.9375,
+ "pos_z": 122,
+ "stations": "Bohr Terminal,Kirby Station,Schumacher Orbital,Goldberg Reach"
+ },
+ {
+ "name": "Chertan",
+ "pos_x": 58.34375,
+ "pos_y": 149.5625,
+ "pos_z": -38.34375,
+ "stations": "Elgin Hub,Lewis Hub,McMullen Vision,Fremion's Inheritance"
+ },
+ {
+ "name": "Chet",
+ "pos_x": 138.6875,
+ "pos_y": -195.65625,
+ "pos_z": 126.46875,
+ "stations": "Amedeo Plana Colony,Nomura Holdings,von Bellingshausen Horizons"
+ },
+ {
+ "name": "Chey",
+ "pos_x": -119.65625,
+ "pos_y": -18.15625,
+ "pos_z": 16.90625,
+ "stations": "Helms Terminal,Clayton Silo,Wiberg Refinery"
+ },
+ {
+ "name": "Cheyenne",
+ "pos_x": -24.65625,
+ "pos_y": 155.84375,
+ "pos_z": -43,
+ "stations": "Hedin Ring,de Caminha Oasis"
+ },
+ {
+ "name": "Chhal",
+ "pos_x": -31.53125,
+ "pos_y": 125.65625,
+ "pos_z": 68.03125,
+ "stations": "Moresby Vision,Isherwood Port,Gregory's Progress"
+ },
+ {
+ "name": "Chhaleda",
+ "pos_x": -125.78125,
+ "pos_y": 69.3125,
+ "pos_z": 91.8125,
+ "stations": "Boulton Terminal,Sturckow Oasis"
+ },
+ {
+ "name": "Chhaleenes",
+ "pos_x": 144.03125,
+ "pos_y": -83.6875,
+ "pos_z": 30.75,
+ "stations": "Marsden Dock,Koishikawa Vision,Fokker Station,Lander Vision,Mozhaysky Dock,Hansen Hub,Cayley Port"
+ },
+ {
+ "name": "Chhalini",
+ "pos_x": 58.9375,
+ "pos_y": -241.25,
+ "pos_z": 59,
+ "stations": "Dufay's Pride"
+ },
+ {
+ "name": "Chi Eridani",
+ "pos_x": 26.28125,
+ "pos_y": -51.75,
+ "pos_z": 4.625,
+ "stations": "Armstrong Mines,Steve Masters,The Ascending Phoenix,Alten Holdings,Berners-Lee Refinery"
+ },
+ {
+ "name": "Chi Herculis",
+ "pos_x": -30.75,
+ "pos_y": 39.71875,
+ "pos_z": 12.78125,
+ "stations": "Bridger Town,Gorbatko Reserve,Gemar Orbital"
+ },
+ {
+ "name": "Chi Nefer",
+ "pos_x": -61.5,
+ "pos_y": 41.1875,
+ "pos_z": 107.25,
+ "stations": "Crown Landing,Wingqvist Outpost"
+ },
+ {
+ "name": "Chi No Ch'orti",
+ "pos_x": 78.90625,
+ "pos_y": -99.0625,
+ "pos_z": 97.46875,
+ "stations": "Hertzsprung Colony,Potocnik Landing,Leberecht Tempel Camp"
+ },
+ {
+ "name": "Chi Nongoi",
+ "pos_x": -123.65625,
+ "pos_y": -114.3125,
+ "pos_z": 40.1875,
+ "stations": "Fabian's Inheritance,Foreman Base"
+ },
+ {
+ "name": "Chi Orionis",
+ "pos_x": 4.3125,
+ "pos_y": -1.0625,
+ "pos_z": -27.90625,
+ "stations": "Carey Terminal,Cayley Terminal"
+ },
+ {
+ "name": "Chi Suix",
+ "pos_x": 36.28125,
+ "pos_y": -154.8125,
+ "pos_z": 24.1875,
+ "stations": "Suzuki City,Backlund Port,Bond Station,Dominique Hub,Hickam Works"
+ },
+ {
+ "name": "Chi-1 Hydrae",
+ "pos_x": 121.46875,
+ "pos_y": 70.28125,
+ "pos_z": 13.5625,
+ "stations": "Armstrong Installation,McClintock Dock,McCandless Beacon"
+ },
+ {
+ "name": "Chia",
+ "pos_x": -131.1875,
+ "pos_y": -112.625,
+ "pos_z": 16.75,
+ "stations": "McKay Installation,Raleigh Point"
+ },
+ {
+ "name": "Chias Vega",
+ "pos_x": -78.5,
+ "pos_y": -1.9375,
+ "pos_z": -115.96875,
+ "stations": "Creamer Dock,Reed Holdings,Ricardo Gateway,Butler Beacon,Faris Platform"
+ },
+ {
+ "name": "Chibiabos",
+ "pos_x": 76.0625,
+ "pos_y": 30,
+ "pos_z": -44.65625,
+ "stations": "Weber Terminal,White's Progress,Stanier Orbital"
+ },
+ {
+ "name": "Chibio",
+ "pos_x": 39.3125,
+ "pos_y": 136.65625,
+ "pos_z": -15.78125,
+ "stations": "Xiaoguan Orbital,Hartsfield Mines,Bethe Ring,Sauma Enterprise"
+ },
+ {
+ "name": "Chibis",
+ "pos_x": 142.96875,
+ "pos_y": 18.125,
+ "pos_z": -83.84375,
+ "stations": "Witt's Progress,Bergerac's Inheritance,Vercors Depot"
+ },
+ {
+ "name": "Chiboneskhe",
+ "pos_x": -109.625,
+ "pos_y": 9.53125,
+ "pos_z": -87.125,
+ "stations": "Aleksandrov Port,Chu City,Al-Battani Dock,Soto Enterprise,Ziemkiewicz Vista"
+ },
+ {
+ "name": "Chiburri",
+ "pos_x": 73.71875,
+ "pos_y": -19.4375,
+ "pos_z": 137.9375,
+ "stations": "Brackett Enterprise,al-Khowarizmi Station,Sekelj Terminal"
+ },
+ {
+ "name": "Chiccan",
+ "pos_x": -11.84375,
+ "pos_y": 120.375,
+ "pos_z": 133.90625,
+ "stations": "Nikitin Colony,Buckell Dock,Beltrami Settlement"
+ },
+ {
+ "name": "Chicchang",
+ "pos_x": 53.46875,
+ "pos_y": -114.53125,
+ "pos_z": 92.96875,
+ "stations": "Sitterly Refinery,Williams Bastion"
+ },
+ {
+ "name": "Chiche",
+ "pos_x": -26.8125,
+ "pos_y": 32.4375,
+ "pos_z": 171.90625,
+ "stations": "Due Depot"
+ },
+ {
+ "name": "Chick Ba",
+ "pos_x": -107.5,
+ "pos_y": -138.71875,
+ "pos_z": -37.0625,
+ "stations": "von Krusenstern Vision,Alvarez de Pineda City,Russ Hub"
+ },
+ {
+ "name": "Chick Bahpu",
+ "pos_x": 90.90625,
+ "pos_y": -153.9375,
+ "pos_z": 35.125,
+ "stations": "Dyson Works"
+ },
+ {
+ "name": "Chick Bara",
+ "pos_x": 124.34375,
+ "pos_y": -95.53125,
+ "pos_z": -5.09375,
+ "stations": "Israel Station,Dalgarno Station,Fernao do Po Laboratory,Grigorovich Gateway"
+ },
+ {
+ "name": "Chick Ek",
+ "pos_x": -22.625,
+ "pos_y": -18.875,
+ "pos_z": 129.59375,
+ "stations": "Wheelock Gateway,Magnus Survey"
+ },
+ {
+ "name": "Chicoana",
+ "pos_x": -35,
+ "pos_y": -23.21875,
+ "pos_z": -110.5,
+ "stations": "Drew Colony,Hale Platform,Andrews' Folly,Nagel Platform"
+ },
+ {
+ "name": "Chicoba",
+ "pos_x": -80.78125,
+ "pos_y": -12.125,
+ "pos_z": 161.4375,
+ "stations": "Barjavel Point"
+ },
+ {
+ "name": "Chicomoztoc",
+ "pos_x": 84.21875,
+ "pos_y": -105.59375,
+ "pos_z": -15.03125,
+ "stations": "Goldschmidt Port,Orbik Orbital,Heinemann Hub,Fabricius Gateway,Farghani Orbital,Stasheff Works,Taylor Market,Lee Port,Ilyushin Port,Brom Dock,Brackett Landing"
+ },
+ {
+ "name": "Chieh",
+ "pos_x": 162.4375,
+ "pos_y": -123.9375,
+ "pos_z": 99.5,
+ "stations": "Brouwer Stop"
+ },
+ {
+ "name": "Chiela",
+ "pos_x": -155.875,
+ "pos_y": 80.6875,
+ "pos_z": -14.6875,
+ "stations": "Moore Base"
+ },
+ {
+ "name": "Chielas",
+ "pos_x": -98.3125,
+ "pos_y": 64.34375,
+ "pos_z": -47.40625,
+ "stations": "Alpers Beacon"
+ },
+ {
+ "name": "Chih Hiner",
+ "pos_x": 48.125,
+ "pos_y": -168.03125,
+ "pos_z": -41.125,
+ "stations": "Auzout Port,Eggen Hub,Ikeya Station,Soukup Town,Kanai Plant"
+ },
+ {
+ "name": "Chih Sungu",
+ "pos_x": -111.84375,
+ "pos_y": -11.9375,
+ "pos_z": -44.71875,
+ "stations": "Ashby Platform,Trevithick Terminal,Dutton Point"
+ },
+ {
+ "name": "Chih Zha",
+ "pos_x": 79.21875,
+ "pos_y": -88.9375,
+ "pos_z": -59.5,
+ "stations": "Hinz Hangar,Valigursky's Folly,Sverdrup Beacon"
+ },
+ {
+ "name": "Chih Zhi",
+ "pos_x": -45.59375,
+ "pos_y": 57.375,
+ "pos_z": 70.4375,
+ "stations": "Hire Terminal,Larson Barracks,Melroy Vision"
+ },
+ {
+ "name": "Chih Zhua",
+ "pos_x": 79.21875,
+ "pos_y": -20.40625,
+ "pos_z": 26.875,
+ "stations": "Anthony Enterprise,Ray Base,Grant's Folly,Walker Orbital,Stiegler Vision"
+ },
+ {
+ "name": "Chiko",
+ "pos_x": 20.5,
+ "pos_y": -146,
+ "pos_z": 131.21875,
+ "stations": "Ferguson Prospect,Mies van der Rohe Colony,Morgan Depot"
+ },
+ {
+ "name": "Chiks",
+ "pos_x": 44.4375,
+ "pos_y": 100.125,
+ "pos_z": -53.9375,
+ "stations": "Curie Refinery,Auer Point"
+ },
+ {
+ "name": "Chimagi",
+ "pos_x": -20.4375,
+ "pos_y": -83.78125,
+ "pos_z": -138.9375,
+ "stations": "Dalton Plant,Jacquard Port"
+ },
+ {
+ "name": "Chimasates",
+ "pos_x": 18.75,
+ "pos_y": -109.71875,
+ "pos_z": 88.75,
+ "stations": "Adragna Terminal,Pittendreigh Vision,Burnham Landing"
+ },
+ {
+ "name": "Chimba",
+ "pos_x": -68.59375,
+ "pos_y": -7.03125,
+ "pos_z": 49.6875,
+ "stations": "Bean Point,Hurley Port,Thornton Hub,Harris Station,Henry Orbital,Nikolayev Hub,Stillman's Folly,Williams Arena,Hambly Point,Hertz Camp"
+ },
+ {
+ "name": "Chimbia",
+ "pos_x": 35.1875,
+ "pos_y": -104.0625,
+ "pos_z": 104.59375,
+ "stations": "Riccioli Colony,Tuttle Orbital,Dorsey Depot"
+ },
+ {
+ "name": "Chimbo",
+ "pos_x": -61.5625,
+ "pos_y": 85.75,
+ "pos_z": 150.15625,
+ "stations": "Andersson Sanctuary,Lukyanenko's Folly"
+ },
+ {
+ "name": "Chime",
+ "pos_x": 114.96875,
+ "pos_y": -50.90625,
+ "pos_z": -82.4375,
+ "stations": "Vasquez de Coronado Asylum"
+ },
+ {
+ "name": "Chimechilo",
+ "pos_x": 20.8125,
+ "pos_y": -84.875,
+ "pos_z": -12.90625,
+ "stations": "Arago Terminal,Townes Port,Porco Dock,Stiegler Landing,Finlay Beacon,Pippin Installation"
+ },
+ {
+ "name": "Chimiri",
+ "pos_x": 24.15625,
+ "pos_y": -53.40625,
+ "pos_z": 99.78125,
+ "stations": "Karachkina Orbital,Rigaux Ring,Harding Dock,Tucker Beacon,Berkey Terminal,Furukawa Orbital,Cerulli Terminal,Kornbluth Vision,Grant Port,Mueller City,Soukup Keep,Lippisch Hub,Schade Horizons"
+ },
+ {
+ "name": "Chin",
+ "pos_x": -0.96875,
+ "pos_y": 66.625,
+ "pos_z": 106.53125,
+ "stations": "Thurston Port,Norman Hub,Key Survey,Wylie Terminal"
+ },
+ {
+ "name": "Chinas",
+ "pos_x": -145.28125,
+ "pos_y": -68.96875,
+ "pos_z": -11.75,
+ "stations": "Sinclair Colony,Compton Bastion,McCormick Station"
+ },
+ {
+ "name": "Chinataxo",
+ "pos_x": -143.6875,
+ "pos_y": 19.34375,
+ "pos_z": 61.8125,
+ "stations": "Neff Arena"
+ },
+ {
+ "name": "Chinautsu",
+ "pos_x": -106.5625,
+ "pos_y": 52.4375,
+ "pos_z": 131.90625,
+ "stations": "Lessing Dock,Franke Barracks"
+ },
+ {
+ "name": "Chinga",
+ "pos_x": 132.21875,
+ "pos_y": -138.78125,
+ "pos_z": 31.9375,
+ "stations": "Wilson Stop"
+ },
+ {
+ "name": "Chingaa",
+ "pos_x": 143.5625,
+ "pos_y": -15.75,
+ "pos_z": -93.4375,
+ "stations": "Hovell Dock"
+ },
+ {
+ "name": "Chinggamin",
+ "pos_x": 168.5625,
+ "pos_y": -156.40625,
+ "pos_z": 44.625,
+ "stations": "Addams Landing,Bass Installation,Thollon Terminal"
+ },
+ {
+ "name": "Chingpho",
+ "pos_x": 170.75,
+ "pos_y": -103.375,
+ "pos_z": 6.15625,
+ "stations": "Edmondson Mines"
+ },
+ {
+ "name": "Chini",
+ "pos_x": 52.75,
+ "pos_y": 43.65625,
+ "pos_z": -31.46875,
+ "stations": "Mallory Dock,Laird Station,Carlisle Works,Dayuan Installation,Janes Enterprise,den Berg's Folly"
+ },
+ {
+ "name": "Chiniani",
+ "pos_x": -79.03125,
+ "pos_y": 35.1875,
+ "pos_z": 128.84375,
+ "stations": "Hume Platform,Slonczewski's Progress"
+ },
+ {
+ "name": "Chinicollo",
+ "pos_x": -42.03125,
+ "pos_y": -34,
+ "pos_z": 65.75,
+ "stations": "Onufriyenko Mine"
+ },
+ {
+ "name": "Chinner",
+ "pos_x": -4.59375,
+ "pos_y": -137.4375,
+ "pos_z": 62.75,
+ "stations": "Mikoyan Station,Leberecht Tempel Terminal,Langley Horizons,Chertovsky Station,Frobenius' Pride"
+ },
+ {
+ "name": "Chino",
+ "pos_x": 34.5625,
+ "pos_y": -106.53125,
+ "pos_z": -27.875,
+ "stations": "Stafford Base,McKie Dock"
+ },
+ {
+ "name": "Chinoharra",
+ "pos_x": 146.4375,
+ "pos_y": -123.625,
+ "pos_z": 37.78125,
+ "stations": "Darwin Dock,Zajdel Depot"
+ },
+ {
+ "name": "Chinouk",
+ "pos_x": -107.90625,
+ "pos_y": -70.375,
+ "pos_z": 56.21875,
+ "stations": "Kwolek Hub,Collins Horizons,Lounge Beacon"
+ },
+ {
+ "name": "Chinovane",
+ "pos_x": 140.25,
+ "pos_y": -165.84375,
+ "pos_z": 80.625,
+ "stations": "Herschel Mines"
+ },
+ {
+ "name": "Chipatisany",
+ "pos_x": 77.15625,
+ "pos_y": -22.0625,
+ "pos_z": 16.4375,
+ "stations": "Morgan Hub,Cowling Hub,Artzybasheff Landing"
+ },
+ {
+ "name": "Chipi",
+ "pos_x": 119.03125,
+ "pos_y": 95.46875,
+ "pos_z": -62.59375,
+ "stations": "Gloss Orbital,Lupoff Terminal,Milnor Relay"
+ },
+ {
+ "name": "Chipiapoos",
+ "pos_x": 63.1875,
+ "pos_y": -20.71875,
+ "pos_z": 42.625,
+ "stations": "Dedman Ring,Cook Orbital,King Depot"
+ },
+ {
+ "name": "Chipibogi",
+ "pos_x": 1.4375,
+ "pos_y": -4.65625,
+ "pos_z": -124.125,
+ "stations": "Tanner Platform"
+ },
+ {
+ "name": "Chique",
+ "pos_x": -102,
+ "pos_y": -39.1875,
+ "pos_z": 61.5625,
+ "stations": "Hahn Outpost,Stephenson Base,Lopez de Villalobos Landing,Birdseye Dock"
+ },
+ {
+ "name": "Chira",
+ "pos_x": 95.28125,
+ "pos_y": -151.71875,
+ "pos_z": -8.1875,
+ "stations": "Dufay Vision,Hereford Prospect"
+ },
+ {
+ "name": "Chirai",
+ "pos_x": 144.4375,
+ "pos_y": -127.90625,
+ "pos_z": 26.90625,
+ "stations": "Derekas Horizons,Armero Depot,Pimi Terminal"
+ },
+ {
+ "name": "Chireni",
+ "pos_x": -26.09375,
+ "pos_y": 19.53125,
+ "pos_z": 138.28125,
+ "stations": "Forest City,Berezin Enterprise"
+ },
+ {
+ "name": "Chirskokum",
+ "pos_x": 98.78125,
+ "pos_y": -59.34375,
+ "pos_z": 84.71875,
+ "stations": "Yakovlev Vision,Hirayama Orbital,Baillaud City,Froude Palace"
+ },
+ {
+ "name": "Chit",
+ "pos_x": 136.90625,
+ "pos_y": -22.0625,
+ "pos_z": -14,
+ "stations": "Laval Mines"
+ },
+ {
+ "name": "Chiut",
+ "pos_x": 90,
+ "pos_y": -127.875,
+ "pos_z": -25.03125,
+ "stations": "Andoyer City,Friend Reach,Plancius City,Sitter Station"
+ },
+ {
+ "name": "Chiutl",
+ "pos_x": 110.03125,
+ "pos_y": -63.1875,
+ "pos_z": 122.96875,
+ "stations": "Bessel Gateway,Gould Terminal,Roggeveen Works,Zenbei Vision"
+ },
+ {
+ "name": "Chlevit",
+ "pos_x": -161.90625,
+ "pos_y": 48.125,
+ "pos_z": -18.21875,
+ "stations": "Leonov City,Baird Orbital,Swigert Survey,Witt Prospect,Pashin's Progress,Hiraga Beacon"
+ },
+ {
+ "name": "Chlevoi",
+ "pos_x": -122.375,
+ "pos_y": -84.625,
+ "pos_z": 100.375,
+ "stations": "Spedding Survey,Perrin Settlement"
+ },
+ {
+ "name": "Chnemine",
+ "pos_x": -138.90625,
+ "pos_y": -74.25,
+ "pos_z": -36.53125,
+ "stations": "Birkhoff Station"
+ },
+ {
+ "name": "Chnouk",
+ "pos_x": -7.0625,
+ "pos_y": -45.125,
+ "pos_z": 60.46875,
+ "stations": "Van Scyoc Horizons,Pytheas Enterprise,Auer Silo"
+ },
+ {
+ "name": "Chnoumis",
+ "pos_x": -79.625,
+ "pos_y": -120.59375,
+ "pos_z": 21.09375,
+ "stations": "Hermite Port,Nachtigal's Inheritance"
+ },
+ {
+ "name": "Chnouphis",
+ "pos_x": -179.9375,
+ "pos_y": 27.71875,
+ "pos_z": 68.3125,
+ "stations": "Crown City"
+ },
+ {
+ "name": "Chnumandr",
+ "pos_x": 24.1875,
+ "pos_y": -99.0625,
+ "pos_z": -59.21875,
+ "stations": "Weston Relay"
+ },
+ {
+ "name": "Chnumar",
+ "pos_x": 107.46875,
+ "pos_y": -170.34375,
+ "pos_z": 70.59375,
+ "stations": "Riazuddin Terminal,d'Allonville Hub,Lomonosov Orbital,Nobel Horizons,Abell Settlement"
+ },
+ {
+ "name": "Chnuphis",
+ "pos_x": -80.3125,
+ "pos_y": 36.96875,
+ "pos_z": 29,
+ "stations": "Shaw Settlement,Nagata Port,Sheckley Settlement,Gidzenko Survey"
+ },
+ {
+ "name": "Chnupi",
+ "pos_x": -47.78125,
+ "pos_y": 119.90625,
+ "pos_z": 18.375,
+ "stations": "McMullen Orbital,Williams Settlement,Burroughs Base,Beebe Plant"
+ },
+ {
+ "name": "Chock",
+ "pos_x": 98,
+ "pos_y": -120.4375,
+ "pos_z": -45.59375,
+ "stations": "Tisserand Hub,Tange Dock,Glass Port,Laird Vision"
+ },
+ {
+ "name": "Chodhara",
+ "pos_x": -35.34375,
+ "pos_y": -10.71875,
+ "pos_z": -165.09375,
+ "stations": "Sullivan Enterprise,Wescott Terminal,Flade Dock,Fung Installation"
+ },
+ {
+ "name": "Chodhna",
+ "pos_x": 27.5,
+ "pos_y": 57.09375,
+ "pos_z": 116.1875,
+ "stations": "Meade Arena,Savery Relay"
+ },
+ {
+ "name": "Choeng Yu",
+ "pos_x": -107.96875,
+ "pos_y": -30,
+ "pos_z": 107.1875,
+ "stations": "Strekalov Landing"
+ },
+ {
+ "name": "Choenpetese",
+ "pos_x": 95.1875,
+ "pos_y": -6.90625,
+ "pos_z": 21.4375,
+ "stations": "Paulmier de Gonneville Installation"
+ },
+ {
+ "name": "Choere",
+ "pos_x": 106.375,
+ "pos_y": 115.6875,
+ "pos_z": -17.25,
+ "stations": "Drew Mines"
+ },
+ {
+ "name": "Choerii",
+ "pos_x": -83.6875,
+ "pos_y": 3.3125,
+ "pos_z": 138.4375,
+ "stations": "Barba Vision"
+ },
+ {
+ "name": "Choerini",
+ "pos_x": -45.375,
+ "pos_y": -101.625,
+ "pos_z": 114.875,
+ "stations": "Beshore Survey"
+ },
+ {
+ "name": "Choki",
+ "pos_x": 128.09375,
+ "pos_y": -5.84375,
+ "pos_z": -94.53125,
+ "stations": "Afanasyev Mine,Sommerfeld Dock,McMonagle Depot"
+ },
+ {
+ "name": "Chokota",
+ "pos_x": 132.53125,
+ "pos_y": 9.8125,
+ "pos_z": 34.84375,
+ "stations": "Ford Enterprise,Afanasyev City,Scheutz Ring,Navigator Base,Asaro Camp"
+ },
+ {
+ "name": "Cholhou",
+ "pos_x": 91.15625,
+ "pos_y": 33.03125,
+ "pos_z": 107.34375,
+ "stations": "Scheerbart Point,Napier Landing,Crown Plant"
+ },
+ {
+ "name": "Cholhynici",
+ "pos_x": 35.15625,
+ "pos_y": 60.0625,
+ "pos_z": -104.65625,
+ "stations": "Wiberg Mine,Wedge Installation"
+ },
+ {
+ "name": "Cholul",
+ "pos_x": 12.96875,
+ "pos_y": -128.71875,
+ "pos_z": 75.21875,
+ "stations": "Hubble Holdings,Penzias Station"
+ },
+ {
+ "name": "Chona",
+ "pos_x": 108.0625,
+ "pos_y": -111.46875,
+ "pos_z": 65.4375,
+ "stations": "Smoot Station,Abbott Vision,Iben Orbital,Medupe Legacy"
+ },
+ {
+ "name": "Chond",
+ "pos_x": 1.78125,
+ "pos_y": 77.625,
+ "pos_z": -64.6875,
+ "stations": "Wilder Enterprise,Kandel Ring"
+ },
+ {
+ "name": "Chong Dungu",
+ "pos_x": 17.375,
+ "pos_y": -156.90625,
+ "pos_z": 94.21875,
+ "stations": "Schlesinger Relay,Lee Camp"
+ },
+ {
+ "name": "Chongguls",
+ "pos_x": -45.0625,
+ "pos_y": 46.9375,
+ "pos_z": 107.40625,
+ "stations": "Divis Terminal,Filipchenko Gateway,Revin Installation,Gerst Port,Meade Installation"
+ },
+ {
+ "name": "Chongi",
+ "pos_x": 72.96875,
+ "pos_y": -91.5625,
+ "pos_z": 149.53125,
+ "stations": "Plancius Hub,Bolton City,Paczynski Port,Kagan Prospect,Alexandrov Landing"
+ },
+ {
+ "name": "Chongloi",
+ "pos_x": 169.46875,
+ "pos_y": -213.28125,
+ "pos_z": 77.59375,
+ "stations": "Asami's Claim"
+ },
+ {
+ "name": "Chongquan",
+ "pos_x": -20.78125,
+ "pos_y": 13.1875,
+ "pos_z": -37.4375,
+ "stations": "Bagian Point,Pippin Terminal"
+ },
+ {
+ "name": "Chono",
+ "pos_x": 72.84375,
+ "pos_y": -185.84375,
+ "pos_z": 61.6875,
+ "stations": "Siddha Ring,Joseph Delambre Terminal,Shklovsky's Progress,Delsanti Vision,Sohl's Inheritance"
+ },
+ {
+ "name": "Chonost",
+ "pos_x": 13.40625,
+ "pos_y": -18.125,
+ "pos_z": -81.28125,
+ "stations": "Savitskaya Hub"
+ },
+ {
+ "name": "Chonpon",
+ "pos_x": 57.03125,
+ "pos_y": 38.5,
+ "pos_z": -79.5,
+ "stations": "Malerba Hub,Snyder Landing,Tani Platform"
+ },
+ {
+ "name": "Chons",
+ "pos_x": 47.3125,
+ "pos_y": -68.53125,
+ "pos_z": -95.9375,
+ "stations": "Budrys Terminal,Lee Station,Barth Enterprise,Bisson Orbital,Zoline Port,Hodgson Dock,Bode Observatory,Alexander Hub"
+ },
+ {
+ "name": "Chonsu",
+ "pos_x": 149.28125,
+ "pos_y": -11.40625,
+ "pos_z": 2.8125,
+ "stations": "Haisheng Refinery,Bartoe Depot,Kovalyonok Terminal,Georg Bothe's Inheritance"
+ },
+ {
+ "name": "Chonta",
+ "pos_x": 151.90625,
+ "pos_y": -200.59375,
+ "pos_z": 48.1875,
+ "stations": "Salpeter Depot"
+ },
+ {
+ "name": "Chontaiko",
+ "pos_x": 123.8125,
+ "pos_y": -14.0625,
+ "pos_z": 66.71875,
+ "stations": "Warner Landing,Waldeck Reach"
+ },
+ {
+ "name": "Chontamenti",
+ "pos_x": -64.9375,
+ "pos_y": 93.28125,
+ "pos_z": -99.125,
+ "stations": "Phillifent Colony,Houtman Landing"
+ },
+ {
+ "name": "Chontantici",
+ "pos_x": 49.8125,
+ "pos_y": 37.96875,
+ "pos_z": 21.84375,
+ "stations": "Rzeppa Dock,Rontgen Beacon,Mourelle Relay"
+ },
+ {
+ "name": "Chonti",
+ "pos_x": 116.71875,
+ "pos_y": -47.84375,
+ "pos_z": 105.40625,
+ "stations": "Anderson Ring,Hadid Vision,Harrington Orbital,Besonders Landing,King Prospect"
+ },
+ {
+ "name": "Chontina",
+ "pos_x": 135.21875,
+ "pos_y": 11.53125,
+ "pos_z": -12.03125,
+ "stations": "Lu's Claim,Ansari Beacon,Zelazny Beacon,McNair's Folly"
+ },
+ {
+ "name": "Chorathia",
+ "pos_x": 138.6875,
+ "pos_y": 3.9375,
+ "pos_z": -38.3125,
+ "stations": "Maclaurin Base,McCulley Orbital,VanderMeer Terminal"
+ },
+ {
+ "name": "Chorote",
+ "pos_x": 108.09375,
+ "pos_y": -13.1875,
+ "pos_z": -23.03125,
+ "stations": "Kondratyev Settlement"
+ },
+ {
+ "name": "Chotec",
+ "pos_x": 82.15625,
+ "pos_y": -2.15625,
+ "pos_z": 51.5,
+ "stations": "von Krusenstern's Progress,Marques Dock,Betancourt Refinery,Davis Dock"
+ },
+ {
+ "name": "Choth",
+ "pos_x": -35.53125,
+ "pos_y": 12.4375,
+ "pos_z": 101.9375,
+ "stations": "Bolotov Gateway"
+ },
+ {
+ "name": "Chothe",
+ "pos_x": -15.75,
+ "pos_y": 18.53125,
+ "pos_z": -87.9375,
+ "stations": "Bartoe Platform,Vishweswarayya Beacon"
+ },
+ {
+ "name": "Chou Wargl",
+ "pos_x": -60.90625,
+ "pos_y": 6.03125,
+ "pos_z": 158.3125,
+ "stations": "Herreshoff Hub,Bernoulli Dock,Hale Vision,Kandel City,Sanger Refinery"
+ },
+ {
+ "name": "Choujem",
+ "pos_x": 23.3125,
+ "pos_y": 32.0625,
+ "pos_z": -137.3125,
+ "stations": "Gromov Orbital,Szentmartony Survey"
+ },
+ {
+ "name": "Choujemait",
+ "pos_x": -114.59375,
+ "pos_y": 3.0625,
+ "pos_z": -40.96875,
+ "stations": "Norman Settlement,Gloss Plant,Mitra Dock,Banks' Pride"
+ },
+ {
+ "name": "Chouvumbia",
+ "pos_x": 98.28125,
+ "pos_y": 56.5625,
+ "pos_z": -81.53125,
+ "stations": "Vogel City,Sevastyanov Dock,Hooke Holdings"
+ },
+ {
+ "name": "Chowa",
+ "pos_x": 48.625,
+ "pos_y": 88.9375,
+ "pos_z": 104.125,
+ "stations": "Constantine Dock,Nikitin Point"
+ },
+ {
+ "name": "Chowei",
+ "pos_x": -20.8125,
+ "pos_y": -8.84375,
+ "pos_z": 46.8125,
+ "stations": "Swigert Port"
+ },
+ {
+ "name": "Chrysus",
+ "pos_x": -9537.9375,
+ "pos_y": -922.1875,
+ "pos_z": 19820.1875,
+ "stations": "Rock"
+ },
+ {
+ "name": "Chu I",
+ "pos_x": -71.5,
+ "pos_y": 89,
+ "pos_z": 21.71875,
+ "stations": "Kinsey Port,Ferguson Port,Forrester Settlement"
+ },
+ {
+ "name": "Chu Jona",
+ "pos_x": -75.0625,
+ "pos_y": 94.875,
+ "pos_z": -57.40625,
+ "stations": "Brillant Dock"
+ },
+ {
+ "name": "Chu Pere",
+ "pos_x": -84.5,
+ "pos_y": -113.8125,
+ "pos_z": -78.25,
+ "stations": "Bendell Ring,Cowper Relay,Ellern Relay"
+ },
+ {
+ "name": "Chualang Mu",
+ "pos_x": -56.1875,
+ "pos_y": -100.90625,
+ "pos_z": 28.5,
+ "stations": "McMullen Installation,Pytheas Point"
+ },
+ {
+ "name": "Chuan Shun",
+ "pos_x": 75.65625,
+ "pos_y": -215.15625,
+ "pos_z": -62.3125,
+ "stations": "Altuna Settlement"
+ },
+ {
+ "name": "Chuanabosso",
+ "pos_x": -100.96875,
+ "pos_y": -0.78125,
+ "pos_z": -99.34375,
+ "stations": "Quick Vision,Ivens Horizons,Irens Mines,Crowley Point"
+ },
+ {
+ "name": "Chuang",
+ "pos_x": 205,
+ "pos_y": -128.78125,
+ "pos_z": -10.375,
+ "stations": "Robert Orbital,van Vogt Gateway"
+ },
+ {
+ "name": "Chuang Kong",
+ "pos_x": 67.84375,
+ "pos_y": -29.40625,
+ "pos_z": 53.125,
+ "stations": "Bok Vision,Debehogne Orbital"
+ },
+ {
+ "name": "Chuang Mu",
+ "pos_x": -103.8125,
+ "pos_y": -43.75,
+ "pos_z": -92.21875,
+ "stations": "Naddoddur Dock,Onnes Base,Soto Survey"
+ },
+ {
+ "name": "Chuangubu",
+ "pos_x": 160.03125,
+ "pos_y": 36.34375,
+ "pos_z": 61.21875,
+ "stations": "West Gateway,Hoshi City,Beaufoy Works"
+ },
+ {
+ "name": "Chuelche",
+ "pos_x": 69.625,
+ "pos_y": -10.875,
+ "pos_z": -8.78125,
+ "stations": "Sellers Relay,Artyukhin Relay,Fuglesang Landing"
+ },
+ {
+ "name": "Chuelchs",
+ "pos_x": 70.15625,
+ "pos_y": 22.125,
+ "pos_z": 40.59375,
+ "stations": "Hopkins Installation,Bertin Holdings,Heyerdahl Horizons"
+ },
+ {
+ "name": "Chuen",
+ "pos_x": 71.21875,
+ "pos_y": -75,
+ "pos_z": -19.59375,
+ "stations": "Harding Dock,Sabine Dock,Shirazi Gateway"
+ },
+ {
+ "name": "Chuencani",
+ "pos_x": -115.9375,
+ "pos_y": 23.75,
+ "pos_z": -141.21875,
+ "stations": "Brunner Platform"
+ },
+ {
+ "name": "Chuevik",
+ "pos_x": -82.5,
+ "pos_y": -63.375,
+ "pos_z": 55,
+ "stations": "Gotlieb's Progress,Prunariu Port,Duffy Point"
+ },
+ {
+ "name": "Chuj",
+ "pos_x": -83.875,
+ "pos_y": 41.5,
+ "pos_z": -156.28125,
+ "stations": "Eskridge Asylum"
+ },
+ {
+ "name": "Chujohim",
+ "pos_x": -78.1875,
+ "pos_y": -57.03125,
+ "pos_z": 74.34375,
+ "stations": "Burnet Hub,Parker Refinery,Oleskiw Depot"
+ },
+ {
+ "name": "Chujohimba",
+ "pos_x": 66.65625,
+ "pos_y": -13.375,
+ "pos_z": 53.5625,
+ "stations": "Mikoyan Hub,Gold Dock,O'Donnell Ring,Wolfe City,Cantor Port,Flynn Port,Ellern Ring,Abasheli Ring,Baird Port,Yu Hub,Kandrup Barracks,Sucharitkul Colony,Carleson Holdings,Bergerac Horizons,Boltzmann's Inheritance,Polya Vision"
+ },
+ {
+ "name": "Chukchair",
+ "pos_x": 90.34375,
+ "pos_y": -141.625,
+ "pos_z": -73.71875,
+ "stations": "Tomita Penal colony,de Seversky Penal colony"
+ },
+ {
+ "name": "Chukchan",
+ "pos_x": 142.65625,
+ "pos_y": 56.46875,
+ "pos_z": 40.34375,
+ "stations": "Chamitoff Station,Redi Hub,Huxley Penal colony,Conti Observatory"
+ },
+ {
+ "name": "Chukchisalk",
+ "pos_x": 63.46875,
+ "pos_y": 97.03125,
+ "pos_z": -40.84375,
+ "stations": "Blalock Terminal,Bose Outpost"
+ },
+ {
+ "name": "Chukchitan",
+ "pos_x": 92.1875,
+ "pos_y": -21.75,
+ "pos_z": -32.125,
+ "stations": "Brandenstein Terminal,Ellis Orbital,Galvani's Progress,Tyurin Dock"
+ },
+ {
+ "name": "Chukchu",
+ "pos_x": -23.71875,
+ "pos_y": -0.71875,
+ "pos_z": 27.0625,
+ "stations": "Chapman Plant,Roberts Platform,Makarov Dock,Herbert Relay"
+ },
+ {
+ "name": "Chuku",
+ "pos_x": 137.625,
+ "pos_y": 45.3125,
+ "pos_z": -32.4375,
+ "stations": "Hahn Terminal,Dedman Station,Thornycroft Port,Wilson Landing,Blish's Inheritance"
+ },
+ {
+ "name": "Chukua",
+ "pos_x": -115.8125,
+ "pos_y": -22.90625,
+ "pos_z": 100.40625,
+ "stations": "Kerwin Point"
+ },
+ {
+ "name": "Chukun",
+ "pos_x": 9.78125,
+ "pos_y": -145.8125,
+ "pos_z": -87.8125,
+ "stations": "Gurney Prospect,Bassford Vision,Benford's Inheritance,Brom Station,Bradley Point"
+ },
+ {
+ "name": "Chukuru",
+ "pos_x": 38.40625,
+ "pos_y": -181.65625,
+ "pos_z": 69.25,
+ "stations": "Engle Horizons,Herzog Survey,Kuchemann Settlement"
+ },
+ {
+ "name": "Chul",
+ "pos_x": 110.09375,
+ "pos_y": 112.53125,
+ "pos_z": 31.875,
+ "stations": "Rocklynne Oasis,Pierres Survey,Lopez de Haro Landing"
+ },
+ {
+ "name": "Chun",
+ "pos_x": -151.96875,
+ "pos_y": -63.65625,
+ "pos_z": 68.625,
+ "stations": "Przhevalsky Dock,Levy Landing,Dias Settlement,Lerman Platform,Fernao do Po Landing"
+ },
+ {
+ "name": "Chun Chehe",
+ "pos_x": -136.125,
+ "pos_y": -31.375,
+ "pos_z": 93.96875,
+ "stations": "Moffitt Beacon,Bishop Landing"
+ },
+ {
+ "name": "Chun Hsi",
+ "pos_x": -13.15625,
+ "pos_y": -59.75,
+ "pos_z": 144.3125,
+ "stations": "Polansky Platform"
+ },
+ {
+ "name": "Chun Pindit",
+ "pos_x": -11.5625,
+ "pos_y": -15.40625,
+ "pos_z": -181.09375,
+ "stations": "Maybury Lab,Jones Port,Samuda Terminal,Cook Installation"
+ },
+ {
+ "name": "Chun Tstar",
+ "pos_x": 108.21875,
+ "pos_y": -11.28125,
+ "pos_z": 60.78125,
+ "stations": "Archambault Terminal,Crampton Gateway,Ivanov Enterprise,Moore Landing,Molina Station,Pauli Enterprise,Barnes Market"
+ },
+ {
+ "name": "Chunja",
+ "pos_x": 60.625,
+ "pos_y": -99.40625,
+ "pos_z": -24.375,
+ "stations": "Boscovich Station,Millosevich Holdings,Patry Prospect,Kowal City"
+ },
+ {
+ "name": "Chuntabon",
+ "pos_x": -137.0625,
+ "pos_y": -12.46875,
+ "pos_z": -70.1875,
+ "stations": "Sekowski Prospect,Doyle Depot"
+ },
+ {
+ "name": "Chuntal",
+ "pos_x": -22.03125,
+ "pos_y": 9.6875,
+ "pos_z": -113.21875,
+ "stations": "Thoreau Settlement,Barsanti Vision"
+ },
+ {
+ "name": "Chunza",
+ "pos_x": -15.375,
+ "pos_y": -148.5625,
+ "pos_z": -1.28125,
+ "stations": "Kempf Enterprise,Gaughan Hub,Hughes Observatory"
+ },
+ {
+ "name": "Chup Kamui",
+ "pos_x": 26.28125,
+ "pos_y": -39.15625,
+ "pos_z": 21.5,
+ "stations": "Huberath Settlement,Novitski's Inheritance,Artemis,Savinykh Platform"
+ },
+ {
+ "name": "Chuquiar",
+ "pos_x": 96.125,
+ "pos_y": -164.46875,
+ "pos_z": -3.78125,
+ "stations": "Dassault Enterprise,Digges Enterprise,Smith Landing"
+ },
+ {
+ "name": "Chuquiba",
+ "pos_x": -3.21875,
+ "pos_y": 115.875,
+ "pos_z": 39.21875,
+ "stations": "Macarthur Gateway,Beckman Port,Engle Terminal,Tarentum Keep"
+ },
+ {
+ "name": "Chuquibamba",
+ "pos_x": -80.28125,
+ "pos_y": 125.03125,
+ "pos_z": 11.59375,
+ "stations": "Bailey Mine"
+ },
+ {
+ "name": "Chuquitanth",
+ "pos_x": -24.71875,
+ "pos_y": 80.0625,
+ "pos_z": 80.90625,
+ "stations": "Virchow Mine,Hooke Legacy"
+ },
+ {
+ "name": "Chuuah",
+ "pos_x": 184.25,
+ "pos_y": -133.25,
+ "pos_z": 43.90625,
+ "stations": "Chadwick Terminal,Steakley Installation,Kopal Platform,Furuta Orbital"
+ },
+ {
+ "name": "Chuuahill",
+ "pos_x": 60.84375,
+ "pos_y": -7,
+ "pos_z": -115.90625,
+ "stations": "Hale's Claim,Kozin Platform,Hamilton Relay,Bulychev Plant"
+ },
+ {
+ "name": "Chuuku",
+ "pos_x": 135.25,
+ "pos_y": -172.75,
+ "pos_z": 100.5,
+ "stations": "Bolton Asylum"
+ },
+ {
+ "name": "Chuvaleni",
+ "pos_x": 49.5,
+ "pos_y": -5.15625,
+ "pos_z": 67.625,
+ "stations": "Yegorov Point"
+ },
+ {
+ "name": "Chuvalungu",
+ "pos_x": -79.15625,
+ "pos_y": -54.84375,
+ "pos_z": 93.0625,
+ "stations": "Doi's Claim,Kurland Beacon,Sekelj Vision"
+ },
+ {
+ "name": "Cians",
+ "pos_x": 119.3125,
+ "pos_y": -47.4375,
+ "pos_z": 77.46875,
+ "stations": "Somayaji Mines,O'Neill Arena"
+ },
+ {
+ "name": "Cibaiawar",
+ "pos_x": 121.40625,
+ "pos_y": -88.0625,
+ "pos_z": 104.46875,
+ "stations": "Hiyya Terminal,Resnick Town,Koontz Prospect"
+ },
+ {
+ "name": "Cibambo",
+ "pos_x": 86.40625,
+ "pos_y": 98.46875,
+ "pos_z": 37.875,
+ "stations": "Regiomontanus Vision,Heyerdahl Reach"
+ },
+ {
+ "name": "Cibandana",
+ "pos_x": -104.8125,
+ "pos_y": -117.03125,
+ "pos_z": 32.375,
+ "stations": "Olivas Station,Rontgen Settlement,Bethke Mines"
+ },
+ {
+ "name": "Cibarci",
+ "pos_x": -59.75,
+ "pos_y": -147.59375,
+ "pos_z": -55.4375,
+ "stations": "Liouville Station,McArthur Silo,Fincke Relay"
+ },
+ {
+ "name": "Cibola",
+ "pos_x": 65.25,
+ "pos_y": -156.4375,
+ "pos_z": 6.1875,
+ "stations": "Sansebar,Dominique Vision,Theodore Kyle"
+ },
+ {
+ "name": "Ciboney",
+ "pos_x": 119.875,
+ "pos_y": -70.8125,
+ "pos_z": -15.96875,
+ "stations": "Antoniadi Orbital,Flaugergues Vision,Clark Holdings"
+ },
+ {
+ "name": "Cigu",
+ "pos_x": -5.78125,
+ "pos_y": -45.8125,
+ "pos_z": 135.4375,
+ "stations": "Markham Platform"
+ },
+ {
+ "name": "Cigua",
+ "pos_x": -32.125,
+ "pos_y": 154.5,
+ "pos_z": 87.875,
+ "stations": "Escobar Platform,Thornycroft Settlement"
+ },
+ {
+ "name": "Cigudates",
+ "pos_x": 131.5,
+ "pos_y": 29.78125,
+ "pos_z": -39.5,
+ "stations": "Belyayev Depot"
+ },
+ {
+ "name": "Cigui",
+ "pos_x": 98.25,
+ "pos_y": -19.5625,
+ "pos_z": -48,
+ "stations": "Clark City"
+ },
+ {
+ "name": "Ciguri",
+ "pos_x": 2.0625,
+ "pos_y": 62.53125,
+ "pos_z": 79.09375,
+ "stations": "Ross Orbital,Matteucci Orbital,Zalyotin Installation,Mills Dock,Gurney Port,Brongniart Dock,Bond Relay,Ferguson Arsenal"
+ },
+ {
+ "name": "Ciguru",
+ "pos_x": -82.40625,
+ "pos_y": 3.875,
+ "pos_z": -8.90625,
+ "stations": "Scalzi Arsenal,Hui Mines"
+ },
+ {
+ "name": "Cilbien Zu",
+ "pos_x": 133.0625,
+ "pos_y": -7.65625,
+ "pos_z": -62.71875,
+ "stations": "Marshall Dock,Ryman Terminal,Burbank Port"
+ },
+ {
+ "name": "Cile",
+ "pos_x": 135.3125,
+ "pos_y": -179.21875,
+ "pos_z": 123.25,
+ "stations": "Frimout Port"
+ },
+ {
+ "name": "Cileni",
+ "pos_x": -114.21875,
+ "pos_y": -2.78125,
+ "pos_z": -49.34375,
+ "stations": "Windt City,Bain Point,Blair Installation"
+ },
+ {
+ "name": "Cileut",
+ "pos_x": 107.75,
+ "pos_y": -49.625,
+ "pos_z": -122.0625,
+ "stations": "Mitropoulos Gateway,King Port,Galiano Silo,Hippalus Observatory"
+ },
+ {
+ "name": "Cimicusha",
+ "pos_x": 137.3125,
+ "pos_y": -65.59375,
+ "pos_z": -85.71875,
+ "stations": "Marth Port"
+ },
+ {
+ "name": "Cimih",
+ "pos_x": 55.96875,
+ "pos_y": -64.09375,
+ "pos_z": 60,
+ "stations": "Sopwith Horizons,Roberts Hub"
+ },
+ {
+ "name": "Cimmerians",
+ "pos_x": 44.375,
+ "pos_y": 68.65625,
+ "pos_z": -128,
+ "stations": "Song Survey,Feynman Hub,Carstensz Beacon,Heng Horizons,Bishop Depot"
+ },
+ {
+ "name": "Cimmerighe",
+ "pos_x": -4.125,
+ "pos_y": -92.6875,
+ "pos_z": 29.46875,
+ "stations": "Coles Dock,Van Scyoc Station,Johnson City,Jolliet Base"
+ },
+ {
+ "name": "Cintani",
+ "pos_x": 114.75,
+ "pos_y": 65.25,
+ "pos_z": -104.875,
+ "stations": "Kennicott Horizons,Lopez de Villalobos Landing,Laumer Installation"
+ },
+ {
+ "name": "Cintices",
+ "pos_x": 44.09375,
+ "pos_y": 0.09375,
+ "pos_z": -90.875,
+ "stations": "Bohm Dock,Clayton Point"
+ },
+ {
+ "name": "Cintuatera",
+ "pos_x": -23,
+ "pos_y": -42.5,
+ "pos_z": 106.34375,
+ "stations": "Nye Settlement,Scott Bastion"
+ },
+ {
+ "name": "Circe",
+ "pos_x": -0.09375,
+ "pos_y": -33.375,
+ "pos_z": -32.4375,
+ "stations": "Nowak Refinery,Hornby Refinery"
+ },
+ {
+ "name": "Circios",
+ "pos_x": -3.9375,
+ "pos_y": 54.5,
+ "pos_z": -37.03125,
+ "stations": "Mullane Gateway,Moore Survey,Conrad Dock,Drexler Hub,Sleator Installation,Loncke Observatory"
+ },
+ {
+ "name": "Cismontani",
+ "pos_x": -133.09375,
+ "pos_y": 66.46875,
+ "pos_z": 96.09375,
+ "stations": "MacLeod Relay,White Settlement,Samokutyayev Mines"
+ },
+ {
+ "name": "Cismonthina",
+ "pos_x": -63.28125,
+ "pos_y": 28.125,
+ "pos_z": 114.5625,
+ "stations": "Weston Settlement"
+ },
+ {
+ "name": "Cizi",
+ "pos_x": -13.46875,
+ "pos_y": 108.59375,
+ "pos_z": -51.375,
+ "stations": "Williams Terminal,Forfait Beacon,Clair Enterprise,Tepper Hub"
+ },
+ {
+ "name": "Cizicna",
+ "pos_x": -9.4375,
+ "pos_y": -205.34375,
+ "pos_z": 49,
+ "stations": "Rigaux Hub,Sweet Outpost"
+ },
+ {
+ "name": "Cizicni",
+ "pos_x": -98.03125,
+ "pos_y": 15.03125,
+ "pos_z": 124.625,
+ "stations": "Pullman Terminal,Payne Vision,Merril Orbital,Carleson Depot,Bunnell Terminal,Windt Vision"
+ },
+ {
+ "name": "CK Arietis",
+ "pos_x": -29.3125,
+ "pos_y": -38.875,
+ "pos_z": -85.21875,
+ "stations": "Crown Orbital,Hooke Port,Knight Point"
+ },
+ {
+ "name": "CL Phoenicis",
+ "pos_x": 27.59375,
+ "pos_y": -136.59375,
+ "pos_z": 47.84375,
+ "stations": "Mayer Ring,Titius Ring"
+ },
+ {
+ "name": "Clarus",
+ "pos_x": -22.03125,
+ "pos_y": 2.9375,
+ "pos_z": -89.46875,
+ "stations": "Lorenz City,Wilmore City,Yegorov Vision,MacLean Gateway"
+ },
+ {
+ "name": "Clayagro",
+ "pos_x": 18.875,
+ "pos_y": 42.65625,
+ "pos_z": -151.78125,
+ "stations": "Asher Mines"
+ },
+ {
+ "name": "Clayahu",
+ "pos_x": 82.96875,
+ "pos_y": -76.0625,
+ "pos_z": 37.21875,
+ "stations": "von Biela Dock,Piano Settlement,Bolton Ring,Gunn Legacy"
+ },
+ {
+ "name": "Clayakarma",
+ "pos_x": -20.5,
+ "pos_y": -4.96875,
+ "pos_z": 60.6875,
+ "stations": "Sinclair Port,Kaleri Gateway,Robinson Camp,Roentgen Gateway,Duke City,Burnham Camp,Kennicott Depot,Milepost Zero"
+ },
+ {
+ "name": "Clee",
+ "pos_x": 31.46875,
+ "pos_y": 20.15625,
+ "pos_z": 125.84375,
+ "stations": "Babcock Orbital,Carr Gateway,Goeschke City,Sharman Station,Samuda Point"
+ },
+ {
+ "name": "Clidna",
+ "pos_x": 100.4375,
+ "pos_y": 106.96875,
+ "pos_z": 51.75,
+ "stations": "Houssay Port,Avicenna Landing"
+ },
+ {
+ "name": "Clido Pi",
+ "pos_x": 130.78125,
+ "pos_y": -130.40625,
+ "pos_z": 46.96875,
+ "stations": "Elcano Installation,Abbot Port,Russo Settlement,Bharadwaj Vision,Iben Terminal"
+ },
+ {
+ "name": "Cliocassi",
+ "pos_x": 62.125,
+ "pos_y": -96.3125,
+ "pos_z": 162.375,
+ "stations": "Mueller Outpost"
+ },
+ {
+ "name": "Clionenses",
+ "pos_x": -31.1875,
+ "pos_y": 35.125,
+ "pos_z": -149.03125,
+ "stations": "Ferguson Platform,Barsanti Landing,Oersted Station,la Cosa Legacy"
+ },
+ {
+ "name": "Clionometes",
+ "pos_x": -78.78125,
+ "pos_y": -62.71875,
+ "pos_z": 77.65625,
+ "stations": "Anthony Station,Hamilton Prospect,Ziemkiewicz Terminal,Fermat Holdings,Selous Ring"
+ },
+ {
+ "name": "Clota",
+ "pos_x": -17.9375,
+ "pos_y": -147.21875,
+ "pos_z": -18.09375,
+ "stations": "Brashear Vision"
+ },
+ {
+ "name": "Clotti",
+ "pos_x": -84.4375,
+ "pos_y": 36.75,
+ "pos_z": -20.28125,
+ "stations": "Redi Dock,Robinson Horizons,O'Connor Hangar"
+ },
+ {
+ "name": "CN Bootis",
+ "pos_x": -6.3125,
+ "pos_y": 65.21875,
+ "pos_z": 24.34375,
+ "stations": "Sellings City,Aldiss Dock,Davis Dock,Pullman Refinery,Dowling's Claim"
+ },
+ {
+ "name": "CN Hydrus",
+ "pos_x": 133.5625,
+ "pos_y": -134.21875,
+ "pos_z": 50.125,
+ "stations": "Bailly Hub,Montrose Beacon,Abel Orbital,Shawl Arena,Bharadwaj's Inheritance,Gaultier de Varennes Prospect"
+ },
+ {
+ "name": "Cnepan",
+ "pos_x": 85.3125,
+ "pos_y": 116.21875,
+ "pos_z": 39.53125,
+ "stations": "Brust Station,Pavlou Platform,Meredith Keep,Brooks Horizons"
+ },
+ {
+ "name": "Cnepawetel",
+ "pos_x": 83.53125,
+ "pos_y": -76.625,
+ "pos_z": -68.34375,
+ "stations": "al-Khayyam Prospect,Wilhelm Freeport"
+ },
+ {
+ "name": "Cnephtha",
+ "pos_x": -127.09375,
+ "pos_y": 52.46875,
+ "pos_z": -76.71875,
+ "stations": "Beatty Dock"
+ },
+ {
+ "name": "Coana",
+ "pos_x": 109.8125,
+ "pos_y": -122.25,
+ "pos_z": 106.9375,
+ "stations": "Wilhelm Holdings,Hertzsprung Camp,Naubakht Depot"
+ },
+ {
+ "name": "Coano",
+ "pos_x": 54.46875,
+ "pos_y": -229.875,
+ "pos_z": 54.09375,
+ "stations": "Zarnecki Survey,West Beacon"
+ },
+ {
+ "name": "Coanoa",
+ "pos_x": 26,
+ "pos_y": -179.46875,
+ "pos_z": 57.3125,
+ "stations": "Zwicky Dock,Banno Prospect,Neujmin Platform"
+ },
+ {
+ "name": "Coastakal",
+ "pos_x": 66.5,
+ "pos_y": -113.4375,
+ "pos_z": 15.15625,
+ "stations": "Dolgov Vision,Gaensler Vision"
+ },
+ {
+ "name": "Coastin",
+ "pos_x": 151.5625,
+ "pos_y": 47.9375,
+ "pos_z": 84.375,
+ "stations": "Marusek Dock,O'Leary Lab"
+ },
+ {
+ "name": "Coastsege",
+ "pos_x": 89.4375,
+ "pos_y": -124,
+ "pos_z": 128.25,
+ "stations": "Akiyama Prospect,Corbusier Platform,Scheerbart Refinery"
+ },
+ {
+ "name": "Cocassetsa",
+ "pos_x": -53.96875,
+ "pos_y": -84.3125,
+ "pos_z": -134.625,
+ "stations": "Shukor Installation"
+ },
+ {
+ "name": "Cochi",
+ "pos_x": 62.4375,
+ "pos_y": 57.5625,
+ "pos_z": -94.78125,
+ "stations": "Issigonis Gateway,Lasswitz Point,Pellegrino Laboratory,Gordon Installation"
+ },
+ {
+ "name": "Cochipati",
+ "pos_x": -18.125,
+ "pos_y": -71.5625,
+ "pos_z": -45.90625,
+ "stations": "Nye Station,Kwolek Port"
+ },
+ {
+ "name": "Cocho",
+ "pos_x": 65.34375,
+ "pos_y": -225.875,
+ "pos_z": 46.9375,
+ "stations": "Bohnhoff Holdings,Oberth Orbital,Tupolev Settlement"
+ },
+ {
+ "name": "Cocijo",
+ "pos_x": 31.3125,
+ "pos_y": -52.625,
+ "pos_z": 19.03125,
+ "stations": "Alkaabi,Weiss Vision,Herrington Enterprise,Harry Moore & Co,Back Hub"
+ },
+ {
+ "name": "Cockaigne",
+ "pos_x": 61.75,
+ "pos_y": -130.15625,
+ "pos_z": -1.71875,
+ "stations": "Ottley's Landing,Bond Barracks"
+ },
+ {
+ "name": "Coco",
+ "pos_x": -102.96875,
+ "pos_y": -32.125,
+ "pos_z": -104.375,
+ "stations": "Ivanov Orbital,Low Silo"
+ },
+ {
+ "name": "Cocontae",
+ "pos_x": -17.125,
+ "pos_y": -57.03125,
+ "pos_z": -91.59375,
+ "stations": "Busch Prospect"
+ },
+ {
+ "name": "Cocopa",
+ "pos_x": 146.65625,
+ "pos_y": 31.28125,
+ "pos_z": -20.125,
+ "stations": "Walter Station,Jett Orbital"
+ },
+ {
+ "name": "Cocoria",
+ "pos_x": -40.46875,
+ "pos_y": -115.5,
+ "pos_z": -42.71875,
+ "stations": "Austin Relay"
+ },
+ {
+ "name": "Cocorix",
+ "pos_x": 67.4375,
+ "pos_y": 79.90625,
+ "pos_z": 19.6875,
+ "stations": "Miletus Dock,Serrao Dock,Bounds Barracks,Auld Holdings,Ponce de Leon Settlement"
+ },
+ {
+ "name": "Cocornsa",
+ "pos_x": 53.125,
+ "pos_y": 127.0625,
+ "pos_z": -14.53125,
+ "stations": "Robinson Platform,Gelfand Vision,Chiang Vision"
+ },
+ {
+ "name": "Cocovicente",
+ "pos_x": 55.6875,
+ "pos_y": -165.5625,
+ "pos_z": 104.625,
+ "stations": "Fairey Dock,Alexeyev Landing,Whitcomb Hub,Piano Port,Atwood Observatory"
+ },
+ {
+ "name": "Cocoyet",
+ "pos_x": 103.15625,
+ "pos_y": -132.65625,
+ "pos_z": 6.90625,
+ "stations": "Goldberg Base,Islam Bastion,Lagerkvist Stop"
+ },
+ {
+ "name": "Codorain",
+ "pos_x": 68.625,
+ "pos_y": 37.46875,
+ "pos_z": 42.625,
+ "stations": "Powers Port,Cormack Hub,Safdie Terminal,Ponce de Leon Keep,Dean Station"
+ },
+ {
+ "name": "Coelaba",
+ "pos_x": -60.375,
+ "pos_y": 79.5625,
+ "pos_z": -100.84375,
+ "stations": "Shatalov Station"
+ },
+ {
+ "name": "Coelacara",
+ "pos_x": 137.75,
+ "pos_y": -55.75,
+ "pos_z": 33.09375,
+ "stations": "Tanaka Prospect,Bond Installation,Sandage Hub"
+ },
+ {
+ "name": "Coelachi",
+ "pos_x": 178.25,
+ "pos_y": -173.6875,
+ "pos_z": 109.8125,
+ "stations": "Korolev Port"
+ },
+ {
+ "name": "Coelans",
+ "pos_x": -133.375,
+ "pos_y": 103.5,
+ "pos_z": 88.40625,
+ "stations": "Schiltberger Platform,Abnett Holdings,Gerlache Dock"
+ },
+ {
+ "name": "Coelatuvean",
+ "pos_x": 6.03125,
+ "pos_y": -78.28125,
+ "pos_z": 19.34375,
+ "stations": "Robert Station,Prandtl Beacon"
+ },
+ {
+ "name": "Coelerni",
+ "pos_x": -16.34375,
+ "pos_y": -106.5,
+ "pos_z": 119.96875,
+ "stations": "al-Kashi Dock"
+ },
+ {
+ "name": "Coelesi",
+ "pos_x": 164.15625,
+ "pos_y": -145.5,
+ "pos_z": -29.375,
+ "stations": "Struzan Terminal,Oja Horizons,Stone Arsenal,Coddington Observatory"
+ },
+ {
+ "name": "Coelrind",
+ "pos_x": 3.0625,
+ "pos_y": 35.28125,
+ "pos_z": 6.96875,
+ "stations": "Feoktistov Orbital"
+ },
+ {
+ "name": "Coeus",
+ "pos_x": -9531.71875,
+ "pos_y": -885.3125,
+ "pos_z": 19839.21875,
+ "stations": "Foster Terminal,Oxley Orbital"
+ },
+ {
+ "name": "Cofalyaqa",
+ "pos_x": -110.53125,
+ "pos_y": 46.5,
+ "pos_z": 36.84375,
+ "stations": "Novitski Prospect"
+ },
+ {
+ "name": "Cofalyawa",
+ "pos_x": 95.09375,
+ "pos_y": -0.5,
+ "pos_z": -20.9375,
+ "stations": "Low Horizons,Dobrovolskiy Point,Rennie Legacy"
+ },
+ {
+ "name": "Cofan",
+ "pos_x": -33.71875,
+ "pos_y": 19.34375,
+ "pos_z": 144.15625,
+ "stations": "Kummer Port,Martin Ring"
+ },
+ {
+ "name": "Cofana",
+ "pos_x": -16.65625,
+ "pos_y": -123,
+ "pos_z": -84.625,
+ "stations": "Dzhanibekov Landing,Wittgenstein Prospect"
+ },
+ {
+ "name": "Col 285 Sector AB-E c12-18",
+ "pos_x": 27.25,
+ "pos_y": -99.3125,
+ "pos_z": 168.9375,
+ "stations": "Hulse Plant,Blaylock Barracks"
+ },
+ {
+ "name": "Col 285 Sector AB-E c12-9",
+ "pos_x": 34.40625,
+ "pos_y": -69.71875,
+ "pos_z": 167.90625,
+ "stations": "Malvern Legacy"
+ },
+ {
+ "name": "Col 285 Sector AF-E b13-5",
+ "pos_x": 140.65625,
+ "pos_y": 16.5625,
+ "pos_z": -83.3125,
+ "stations": "Pelt Base,Vasquez de Coronado Settlement"
+ },
+ {
+ "name": "Col 285 Sector AG-O d6-123",
+ "pos_x": -45.34375,
+ "pos_y": -64.0625,
+ "pos_z": 188.8125,
+ "stations": "Block Mine"
+ },
+ {
+ "name": "Col 285 Sector AG-O d6-125",
+ "pos_x": -20.6875,
+ "pos_y": -83.75,
+ "pos_z": 155.1875,
+ "stations": "Shiner Vision"
+ },
+ {
+ "name": "Col 285 Sector AG-O d6-83",
+ "pos_x": -33.21875,
+ "pos_y": -71.28125,
+ "pos_z": 189.65625,
+ "stations": "Haarsma Terminal"
+ },
+ {
+ "name": "Col 285 Sector AG-O d6-95",
+ "pos_x": -7.125,
+ "pos_y": -74.53125,
+ "pos_z": 144.0625,
+ "stations": "Robinson Installation"
+ },
+ {
+ "name": "Col 285 Sector AG-O d6-98",
+ "pos_x": -22.625,
+ "pos_y": -65.125,
+ "pos_z": 159.8125,
+ "stations": "Schmitz Vision"
+ },
+ {
+ "name": "Col 285 Sector AH-K c9-13",
+ "pos_x": -119.125,
+ "pos_y": 96.46875,
+ "pos_z": 32,
+ "stations": "Pook Base"
+ },
+ {
+ "name": "Col 285 Sector AH-K c9-4",
+ "pos_x": -139.9375,
+ "pos_y": 106.96875,
+ "pos_z": 53.84375,
+ "stations": "Vinge Sanctuary"
+ },
+ {
+ "name": "Col 285 Sector AH-K c9-5",
+ "pos_x": -139.375,
+ "pos_y": 101.65625,
+ "pos_z": 52.53125,
+ "stations": "Tavernier's Pride"
+ },
+ {
+ "name": "Col 285 Sector AH-K c9-7",
+ "pos_x": -113.96875,
+ "pos_y": 134.90625,
+ "pos_z": 16.59375,
+ "stations": "Kimberlin Vista"
+ },
+ {
+ "name": "Col 285 Sector AQ-C b14-4",
+ "pos_x": 96.125,
+ "pos_y": 47.59375,
+ "pos_z": -48.25,
+ "stations": "Lynn Settlement,Flint Point,Galouye Mines"
+ },
+ {
+ "name": "Col 285 Sector AQ-P d5-52",
+ "pos_x": -12.1875,
+ "pos_y": -207.25,
+ "pos_z": 123.375,
+ "stations": "McCarthy Base"
+ },
+ {
+ "name": "Col 285 Sector AR-I b11-0",
+ "pos_x": -12.625,
+ "pos_y": 124.53125,
+ "pos_z": -124,
+ "stations": "Weil Survey"
+ },
+ {
+ "name": "Col 285 Sector AY-N b21-2",
+ "pos_x": -129.84375,
+ "pos_y": -7.25,
+ "pos_z": 112.21875,
+ "stations": "Boole Base,Stapledon Depot,Brunner Laboratory"
+ },
+ {
+ "name": "Col 285 Sector AY-N b21-4",
+ "pos_x": -132.9375,
+ "pos_y": -5.03125,
+ "pos_z": 96.53125,
+ "stations": "Garfinkle's Inheritance,Lundwall Prospect"
+ },
+ {
+ "name": "Col 285 Sector AZ-B a29-0",
+ "pos_x": 161.8125,
+ "pos_y": -29.65625,
+ "pos_z": -43.375,
+ "stations": "Morris Hub"
+ },
+ {
+ "name": "Col 285 Sector BA-E b13-7",
+ "pos_x": 128.125,
+ "pos_y": -3.03125,
+ "pos_z": -74.8125,
+ "stations": "Aubakirov Horizons"
+ },
+ {
+ "name": "Col 285 Sector BA-P c6-19",
+ "pos_x": 157.46875,
+ "pos_y": 9.78125,
+ "pos_z": -103.90625,
+ "stations": "Hahn Holdings"
+ },
+ {
+ "name": "Col 285 Sector BA-P c6-23",
+ "pos_x": 155.875,
+ "pos_y": -22.09375,
+ "pos_z": -80.90625,
+ "stations": "Maine Observatory"
+ },
+ {
+ "name": "Col 285 Sector BA-P c6-28",
+ "pos_x": 159.125,
+ "pos_y": -20.78125,
+ "pos_z": -75.3125,
+ "stations": "Hahn Escape"
+ },
+ {
+ "name": "Col 285 Sector BB-E c12-5",
+ "pos_x": 62.75,
+ "pos_y": -101.125,
+ "pos_z": 168.65625,
+ "stations": "Cyrrhus' Pride"
+ },
+ {
+ "name": "Col 285 Sector BB-O c6-10",
+ "pos_x": -235,
+ "pos_y": -195.0625,
+ "pos_z": -75.03125,
+ "stations": "Shatner Prospect"
+ },
+ {
+ "name": "Col 285 Sector BB-O c6-3",
+ "pos_x": -233.1875,
+ "pos_y": -195.96875,
+ "pos_z": -90.4375,
+ "stations": "Brooks Vista"
+ },
+ {
+ "name": "Col 285 Sector BC-K c9-18",
+ "pos_x": -163.375,
+ "pos_y": 75.5,
+ "pos_z": 17.84375,
+ "stations": "Nobel Settlement"
+ },
+ {
+ "name": "Col 285 Sector BC-K c9-6",
+ "pos_x": -161.90625,
+ "pos_y": 68,
+ "pos_z": 37.78125,
+ "stations": "Joliot-Curie Vision,Chorel's Inheritance,Nowak's Folly"
+ },
+ {
+ "name": "Col 285 Sector BF-W a45-0",
+ "pos_x": -31.75,
+ "pos_y": -28.03125,
+ "pos_z": 142.90625,
+ "stations": "Card Terminal,Hurley Relay"
+ },
+ {
+ "name": "Col 285 Sector BG-O d6-129",
+ "pos_x": 91.15625,
+ "pos_y": -87.09375,
+ "pos_z": 183.5625,
+ "stations": "Sterling Survey"
+ },
+ {
+ "name": "Col 285 Sector BG-O d6-91",
+ "pos_x": 73.75,
+ "pos_y": -41.875,
+ "pos_z": 158.625,
+ "stations": "Cowell Hub"
+ },
+ {
+ "name": "Col 285 Sector BG-O d6-93",
+ "pos_x": 45.78125,
+ "pos_y": -83.84375,
+ "pos_z": 161.90625,
+ "stations": "Rond d'Alembert Holdings,Janifer Laboratory"
+ },
+ {
+ "name": "Col 285 Sector BI-P b20-5",
+ "pos_x": -107.21875,
+ "pos_y": -63.28125,
+ "pos_z": 80.1875,
+ "stations": "Gulyaev Terminal"
+ },
+ {
+ "name": "Col 285 Sector BO-M b22-8",
+ "pos_x": -118.71875,
+ "pos_y": 18.96875,
+ "pos_z": 127.5,
+ "stations": "Andree Camp"
+ },
+ {
+ "name": "Col 285 Sector BO-U b18-3",
+ "pos_x": -115.0625,
+ "pos_y": 124.4375,
+ "pos_z": 39.96875,
+ "stations": "Bester Base"
+ },
+ {
+ "name": "Col 285 Sector BQ-N c7-21",
+ "pos_x": 149.5,
+ "pos_y": 84.59375,
+ "pos_z": -54.625,
+ "stations": "Zajdel's Inheritance"
+ },
+ {
+ "name": "Col 285 Sector BT-V b17-2",
+ "pos_x": -157.84375,
+ "pos_y": 59.21875,
+ "pos_z": 31.0625,
+ "stations": "Garnier Relay,Carpini Depot"
+ },
+ {
+ "name": "Col 285 Sector CA-E b13-4",
+ "pos_x": 140.75,
+ "pos_y": 0.53125,
+ "pos_z": -75.71875,
+ "stations": "McDonald Enterprise"
+ },
+ {
+ "name": "Col 285 Sector CC-H b12-1",
+ "pos_x": -24.8125,
+ "pos_y": 139.78125,
+ "pos_z": -98.0625,
+ "stations": "Gann Bastion"
+ },
+ {
+ "name": "Col 285 Sector CC-K c9-20",
+ "pos_x": -133.5625,
+ "pos_y": 87.4375,
+ "pos_z": 42.28125,
+ "stations": "Salak Beacon"
+ },
+ {
+ "name": "Col 285 Sector CH-K c9-17",
+ "pos_x": -63.0625,
+ "pos_y": 127.21875,
+ "pos_z": 19.28125,
+ "stations": "Kennan Observatory"
+ },
+ {
+ "name": "Col 285 Sector CI-N b22-4",
+ "pos_x": 64.15625,
+ "pos_y": 99.625,
+ "pos_z": 128.59375,
+ "stations": "Butler Landing"
+ },
+ {
+ "name": "Col 285 Sector CT-V b17-4",
+ "pos_x": -126.90625,
+ "pos_y": 68.5,
+ "pos_z": 21.375,
+ "stations": "Coulter Forum"
+ },
+ {
+ "name": "Col 285 Sector CW-D c12-19",
+ "pos_x": 28.15625,
+ "pos_y": -118.28125,
+ "pos_z": 161.46875,
+ "stations": "Emshwiller Enterprise"
+ },
+ {
+ "name": "Col 285 Sector CY-N b21-0",
+ "pos_x": -102.34375,
+ "pos_y": -22.125,
+ "pos_z": 100.75,
+ "stations": "Eudoxus Silo,Roberts Prospect"
+ },
+ {
+ "name": "Col 285 Sector DD-P b20-4",
+ "pos_x": -118.625,
+ "pos_y": -80.28125,
+ "pos_z": 92.84375,
+ "stations": "Merchiston's Inheritance,Jiushao Depot"
+ },
+ {
+ "name": "Col 285 Sector DI-P b20-2",
+ "pos_x": -78.84375,
+ "pos_y": -61.75,
+ "pos_z": 86.15625,
+ "stations": "Levi-Civita Horizons,Fawcett Mine"
+ },
+ {
+ "name": "Col 285 Sector DJ-M b22-2",
+ "pos_x": -113.34375,
+ "pos_y": 5.78125,
+ "pos_z": 125.25,
+ "stations": "Patrick Colony"
+ },
+ {
+ "name": "Col 285 Sector DM-K c9-10",
+ "pos_x": 83.375,
+ "pos_y": 174.90625,
+ "pos_z": 39.84375,
+ "stations": "Wood Legacy"
+ },
+ {
+ "name": "Col 285 Sector DM-K c9-11",
+ "pos_x": 77.5625,
+ "pos_y": 170.40625,
+ "pos_z": 41.125,
+ "stations": "Jeury Vision"
+ },
+ {
+ "name": "Col 285 Sector DO-V b17-1",
+ "pos_x": -163.8125,
+ "pos_y": 36.3125,
+ "pos_z": 15.3125,
+ "stations": "Baxter Arsenal"
+ },
+ {
+ "name": "Col 285 Sector DT-U b18-1",
+ "pos_x": -38.4375,
+ "pos_y": 153.25,
+ "pos_z": 35.34375,
+ "stations": "Howe's Claim"
+ },
+ {
+ "name": "Col 285 Sector DV-O c6-6",
+ "pos_x": 156.46875,
+ "pos_y": -53.90625,
+ "pos_z": -91.96875,
+ "stations": "Carr's Inheritance"
+ },
+ {
+ "name": "Col 285 Sector DX-J c9-14",
+ "pos_x": -174.8125,
+ "pos_y": 45.90625,
+ "pos_z": 32.4375,
+ "stations": "Shosuke Barracks"
+ },
+ {
+ "name": "Col 285 Sector DX-J c9-17",
+ "pos_x": -178.15625,
+ "pos_y": 37.46875,
+ "pos_z": 33.75,
+ "stations": "Pinto Vision"
+ },
+ {
+ "name": "Col 285 Sector EB-B b15-4",
+ "pos_x": 143.0625,
+ "pos_y": 55.6875,
+ "pos_z": -43.0625,
+ "stations": "Herjulfsson's Progress,Kimberlin Laboratory"
+ },
+ {
+ "name": "Col 285 Sector EB-O d6-69",
+ "pos_x": 156.71875,
+ "pos_y": -118.09375,
+ "pos_z": 147.15625,
+ "stations": "Bok Base,Vance Beacon"
+ },
+ {
+ "name": "Col 285 Sector EC-H b12-1",
+ "pos_x": 21.28125,
+ "pos_y": 154.46875,
+ "pos_z": -98.03125,
+ "stations": "Brooks Settlement"
+ },
+ {
+ "name": "Col 285 Sector EE-L b23-2",
+ "pos_x": -61.65625,
+ "pos_y": 71.125,
+ "pos_z": 137.84375,
+ "stations": "Vercors Stop"
+ },
+ {
+ "name": "Col 285 Sector EG-N c7-12",
+ "pos_x": 127.4375,
+ "pos_y": -19.21875,
+ "pos_z": -64.78125,
+ "stations": "Taylor Reach,Gordon Depot"
+ },
+ {
+ "name": "Col 285 Sector EG-N c7-22",
+ "pos_x": 124.90625,
+ "pos_y": -18.40625,
+ "pos_z": -29.46875,
+ "stations": "Tall Terminal,Giles Prospect"
+ },
+ {
+ "name": "Col 285 Sector EH-I b11-2",
+ "pos_x": -7.09375,
+ "pos_y": 89.59375,
+ "pos_z": -116.375,
+ "stations": "Franklin Holdings,Adams Horizons"
+ },
+ {
+ "name": "Col 285 Sector EH-Q b20-6",
+ "pos_x": 137.03125,
+ "pos_y": 52.03125,
+ "pos_z": 87.4375,
+ "stations": "Sleator Point,Vlamingh's Progress,Alvares Reach"
+ },
+ {
+ "name": "Col 285 Sector EI-G b12-2",
+ "pos_x": -135.0625,
+ "pos_y": 66.84375,
+ "pos_z": -90.28125,
+ "stations": "Dall Beacon"
+ },
+ {
+ "name": "Col 285 Sector EN-I c10-12",
+ "pos_x": -116.21875,
+ "pos_y": 115.34375,
+ "pos_z": 86.03125,
+ "stations": "McMahon Vista"
+ },
+ {
+ "name": "Col 285 Sector EN-I c10-13",
+ "pos_x": -121.75,
+ "pos_y": 113.875,
+ "pos_z": 84.09375,
+ "stations": "Morris Bastion"
+ },
+ {
+ "name": "Col 285 Sector EN-I c10-2",
+ "pos_x": -107.5625,
+ "pos_y": 96.15625,
+ "pos_z": 73.6875,
+ "stations": "Bujold Barracks,Leinster Landing"
+ },
+ {
+ "name": "Col 285 Sector EN-W b17-1",
+ "pos_x": 74.46875,
+ "pos_y": 154.75,
+ "pos_z": 16.59375,
+ "stations": "Oersted Colony"
+ },
+ {
+ "name": "Col 285 Sector EO-N b21-4",
+ "pos_x": -144.8125,
+ "pos_y": -52.21875,
+ "pos_z": 98.65625,
+ "stations": "Quick Landing"
+ },
+ {
+ "name": "Col 285 Sector EO-N b21-6",
+ "pos_x": -132.875,
+ "pos_y": -57,
+ "pos_z": 95.0625,
+ "stations": "Merchiston Beacon"
+ },
+ {
+ "name": "Col 285 Sector ES-I c10-10",
+ "pos_x": -50.84375,
+ "pos_y": 159.28125,
+ "pos_z": 80.0625,
+ "stations": "Doi Enterprise"
+ },
+ {
+ "name": "Col 285 Sector ES-I c10-13",
+ "pos_x": -46.75,
+ "pos_y": 135.53125,
+ "pos_z": 89.03125,
+ "stations": "Gaspar de Portola Relay"
+ },
+ {
+ "name": "Col 285 Sector ES-I c10-9",
+ "pos_x": -51.5625,
+ "pos_y": 164.03125,
+ "pos_z": 57.25,
+ "stations": "Weyl Survey"
+ },
+ {
+ "name": "Col 285 Sector ET-U b18-1",
+ "pos_x": -20.78125,
+ "pos_y": 139.59375,
+ "pos_z": 45.34375,
+ "stations": "Sturckow Base,Burke Vision"
+ },
+ {
+ "name": "Col 285 Sector EV-D b13-1",
+ "pos_x": 143.40625,
+ "pos_y": -10.65625,
+ "pos_z": -65.8125,
+ "stations": "Clauss Installation,Zettel Keep"
+ },
+ {
+ "name": "Col 285 Sector EZ-K b23-0",
+ "pos_x": -102.03125,
+ "pos_y": 36.90625,
+ "pos_z": 135.125,
+ "stations": "Clough Refinery"
+ },
+ {
+ "name": "Col 285 Sector FB-K b10-1",
+ "pos_x": 88.59375,
+ "pos_y": 78.375,
+ "pos_z": -132.125,
+ "stations": "Beekman Survey,Cousin Enterprise"
+ },
+ {
+ "name": "Col 285 Sector FC-Y b16-1",
+ "pos_x": 127.75,
+ "pos_y": 128.1875,
+ "pos_z": 11,
+ "stations": "Butler Keep"
+ },
+ {
+ "name": "Col 285 Sector FD-N b22-5",
+ "pos_x": 75.40625,
+ "pos_y": 76.03125,
+ "pos_z": 129.15625,
+ "stations": "Ricci's Progress"
+ },
+ {
+ "name": "Col 285 Sector FG-N c7-18",
+ "pos_x": 167.75,
+ "pos_y": -9.0625,
+ "pos_z": -30.4375,
+ "stations": "Robinson Reach"
+ },
+ {
+ "name": "Col 285 Sector FG-N c7-26",
+ "pos_x": 167.75,
+ "pos_y": -19.3125,
+ "pos_z": -53.25,
+ "stations": "Sellers Enterprise"
+ },
+ {
+ "name": "Col 285 Sector FG-N c7-30",
+ "pos_x": 166.09375,
+ "pos_y": 2.1875,
+ "pos_z": -35.15625,
+ "stations": "Cady Settlement"
+ },
+ {
+ "name": "Col 285 Sector FG-N c7-32",
+ "pos_x": 169.25,
+ "pos_y": 0.8125,
+ "pos_z": -39.90625,
+ "stations": "Archimedes Settlement,Schwann Camp"
+ },
+ {
+ "name": "Col 285 Sector FH-I b11-1",
+ "pos_x": 5.8125,
+ "pos_y": 94.15625,
+ "pos_z": -110.21875,
+ "stations": "West Holdings"
+ },
+ {
+ "name": "Col 285 Sector FH-I b11-2",
+ "pos_x": 9.25,
+ "pos_y": 91.09375,
+ "pos_z": -109.84375,
+ "stations": "Norton's Folly,Humphreys Vision,Bohnhoff's Progress"
+ },
+ {
+ "name": "Col 285 Sector FI-I c10-20",
+ "pos_x": -147.53125,
+ "pos_y": 57.65625,
+ "pos_z": 69.96875,
+ "stations": "Stirling Enterprise"
+ },
+ {
+ "name": "Col 285 Sector FI-I c10-21",
+ "pos_x": -146.84375,
+ "pos_y": 67.25,
+ "pos_z": 77.71875,
+ "stations": "Finney Gateway"
+ },
+ {
+ "name": "Col 285 Sector FI-I c10-4",
+ "pos_x": -155.46875,
+ "pos_y": 67.625,
+ "pos_z": 79.5,
+ "stations": "Clair Installation"
+ },
+ {
+ "name": "Col 285 Sector FM-I b11-1",
+ "pos_x": 47.9375,
+ "pos_y": 99.8125,
+ "pos_z": -108.71875,
+ "stations": "Garcia Barracks,Lindbohm Survey"
+ },
+ {
+ "name": "Col 285 Sector FN-I c10-17",
+ "pos_x": -70.25,
+ "pos_y": 124.59375,
+ "pos_z": 86.0625,
+ "stations": "Clayton Base"
+ },
+ {
+ "name": "Col 285 Sector FO-N b21-5",
+ "pos_x": -113.3125,
+ "pos_y": -53.34375,
+ "pos_z": 98.84375,
+ "stations": "Stefanyshyn-Piper Reach"
+ },
+ {
+ "name": "Col 285 Sector FO-N b21-8",
+ "pos_x": -121.625,
+ "pos_y": -59.9375,
+ "pos_z": 107.40625,
+ "stations": "Santos Reach,Henslow Base,Ogden Installation"
+ },
+ {
+ "name": "Col 285 Sector FS-I c10-11",
+ "pos_x": 0.78125,
+ "pos_y": 162.15625,
+ "pos_z": 58.65625,
+ "stations": "Grabe Gateway"
+ },
+ {
+ "name": "Col 285 Sector FS-I c10-5",
+ "pos_x": -7.09375,
+ "pos_y": 170.1875,
+ "pos_z": 93.03125,
+ "stations": "Lawhead Hub"
+ },
+ {
+ "name": "Col 285 Sector FS-J c9-9",
+ "pos_x": -156.3125,
+ "pos_y": 12.875,
+ "pos_z": 51.78125,
+ "stations": "Gurney Beacon,Barentsz Base,Vinge Relay"
+ },
+ {
+ "name": "Col 285 Sector FY-O b20-3",
+ "pos_x": -121,
+ "pos_y": -96.65625,
+ "pos_z": 89.90625,
+ "stations": "Varley Mines,Tucker Bastion"
+ },
+ {
+ "name": "Col 285 Sector FY-O b20-6",
+ "pos_x": -117.03125,
+ "pos_y": -96.125,
+ "pos_z": 83.28125,
+ "stations": "Hilbert Base"
+ },
+ {
+ "name": "Col 285 Sector GC-Q b20-5",
+ "pos_x": 148.71875,
+ "pos_y": 21.9375,
+ "pos_z": 86.21875,
+ "stations": "Krylov Horizons,Asire Base"
+ },
+ {
+ "name": "Col 285 Sector GE-L b23-4",
+ "pos_x": -18.25,
+ "pos_y": 55.96875,
+ "pos_z": 144.65625,
+ "stations": "Clerk Gateway"
+ },
+ {
+ "name": "Col 285 Sector GE-M b22-6",
+ "pos_x": -98.03125,
+ "pos_y": -23.09375,
+ "pos_z": 123.5625,
+ "stations": "Harper Depot"
+ },
+ {
+ "name": "Col 285 Sector GE-T b19-3",
+ "pos_x": -18.1875,
+ "pos_y": 164.5625,
+ "pos_z": 64.0625,
+ "stations": "Drebbel Hub"
+ },
+ {
+ "name": "Col 285 Sector GH-K c9-12",
+ "pos_x": 130.4375,
+ "pos_y": 130.8125,
+ "pos_z": 20.1875,
+ "stations": "Stephenson's Folly"
+ },
+ {
+ "name": "Col 285 Sector GI-I c10-21",
+ "pos_x": -136.15625,
+ "pos_y": 57.71875,
+ "pos_z": 85.09375,
+ "stations": "Kolmogorov Holdings"
+ },
+ {
+ "name": "Col 285 Sector GI-Q b19-1",
+ "pos_x": -103.25,
+ "pos_y": -138.59375,
+ "pos_z": 60,
+ "stations": "Peary Installation"
+ },
+ {
+ "name": "Col 285 Sector GI-W b17-2",
+ "pos_x": 69.21875,
+ "pos_y": 128.1875,
+ "pos_z": 21.34375,
+ "stations": "McHugh Arena,Fox Prospect"
+ },
+ {
+ "name": "Col 285 Sector GN-I c10-14",
+ "pos_x": -45.5,
+ "pos_y": 105.21875,
+ "pos_z": 84.9375,
+ "stations": "Disch Camp,Henderson Vision"
+ },
+ {
+ "name": "Col 285 Sector GU-S b19-2",
+ "pos_x": -96.375,
+ "pos_y": 120.65625,
+ "pos_z": 58.84375,
+ "stations": "Grijalva Installation"
+ },
+ {
+ "name": "Col 285 Sector HB-C b14-4",
+ "pos_x": 133.5625,
+ "pos_y": -11.25,
+ "pos_z": -51.875,
+ "stations": "Abernathy Penal colony,Madsen Prospect,Abbott Horizons"
+ },
+ {
+ "name": "Col 285 Sector HB-N c7-11",
+ "pos_x": 155.53125,
+ "pos_y": -58.34375,
+ "pos_z": -62.5,
+ "stations": "Foglio Silo"
+ },
+ {
+ "name": "Col 285 Sector HC-U d3-48",
+ "pos_x": -84.25,
+ "pos_y": 172.09375,
+ "pos_z": -42.21875,
+ "stations": "Low Works"
+ },
+ {
+ "name": "Col 285 Sector HC-U d3-61",
+ "pos_x": -71.4375,
+ "pos_y": 185.28125,
+ "pos_z": -67.03125,
+ "stations": "MacLeod Beacon,Ordway Penal colony"
+ },
+ {
+ "name": "Col 285 Sector HD-I c10-12",
+ "pos_x": -183.875,
+ "pos_y": 44.75,
+ "pos_z": 79.9375,
+ "stations": "McKee Beacon"
+ },
+ {
+ "name": "Col 285 Sector HD-I c10-13",
+ "pos_x": -181.53125,
+ "pos_y": 42.15625,
+ "pos_z": 80.1875,
+ "stations": "Fowler Mines"
+ },
+ {
+ "name": "Col 285 Sector HD-I c10-14",
+ "pos_x": -166.15625,
+ "pos_y": 41.0625,
+ "pos_z": 69.09375,
+ "stations": "Houtman's Pride"
+ },
+ {
+ "name": "Col 285 Sector HD-I c10-15",
+ "pos_x": -155.15625,
+ "pos_y": 45.9375,
+ "pos_z": 73.46875,
+ "stations": "Thuot Port"
+ },
+ {
+ "name": "Col 285 Sector HD-I c10-6",
+ "pos_x": -163.0625,
+ "pos_y": 19.90625,
+ "pos_z": 72.21875,
+ "stations": "Hertz Depot,Maudslay Lab"
+ },
+ {
+ "name": "Col 285 Sector HG-C b14-1",
+ "pos_x": 155.375,
+ "pos_y": -3.5625,
+ "pos_z": -58.71875,
+ "stations": "Gelfand Prospect,Padalka Keep"
+ },
+ {
+ "name": "Col 285 Sector HN-I c10-17",
+ "pos_x": -19.125,
+ "pos_y": 117.0625,
+ "pos_z": 81.59375,
+ "stations": "Monge Base"
+ },
+ {
+ "name": "Col 285 Sector HN-I c10-19",
+ "pos_x": 12.28125,
+ "pos_y": 128.375,
+ "pos_z": 82.625,
+ "stations": "Fisher Prospect,Shiras Settlement"
+ },
+ {
+ "name": "Col 285 Sector HR-V d2-58",
+ "pos_x": 39.75,
+ "pos_y": 81,
+ "pos_z": -123.6875,
+ "stations": "Carpenter Point,Weber Works"
+ },
+ {
+ "name": "Col 285 Sector HR-V d2-70",
+ "pos_x": 18.40625,
+ "pos_y": 132,
+ "pos_z": -150.96875,
+ "stations": "Resnick Palace"
+ },
+ {
+ "name": "Col 285 Sector HS-G b12-1",
+ "pos_x": 10.15625,
+ "pos_y": 109.375,
+ "pos_z": -105,
+ "stations": "Farrukh's Inheritance,van Vogt Relay"
+ },
+ {
+ "name": "Col 285 Sector HS-I c10-6",
+ "pos_x": 56.59375,
+ "pos_y": 140.84375,
+ "pos_z": 78.59375,
+ "stations": "Lem Mine"
+ },
+ {
+ "name": "Col 285 Sector HZ-K b23-3",
+ "pos_x": -44.40625,
+ "pos_y": 48.75,
+ "pos_z": 144.4375,
+ "stations": "Acharya Holdings"
+ },
+ {
+ "name": "Col 285 Sector HZ-S b19-0",
+ "pos_x": -42.125,
+ "pos_y": 140.34375,
+ "pos_z": 55.90625,
+ "stations": "Shumil Prospect"
+ },
+ {
+ "name": "Col 285 Sector IC-U d3-53",
+ "pos_x": 13.09375,
+ "pos_y": 196.46875,
+ "pos_z": -26.875,
+ "stations": "Allen's Progress"
+ },
+ {
+ "name": "Col 285 Sector IC-U d3-58",
+ "pos_x": -53.5,
+ "pos_y": 197.90625,
+ "pos_z": -29.09375,
+ "stations": "Salak Prospect"
+ },
+ {
+ "name": "Col 285 Sector ID-I c10-10",
+ "pos_x": -122.1875,
+ "pos_y": 47.0625,
+ "pos_z": 56.96875,
+ "stations": "Wang Point"
+ },
+ {
+ "name": "Col 285 Sector ID-I c10-15",
+ "pos_x": -128.5625,
+ "pos_y": 50.1875,
+ "pos_z": 67.53125,
+ "stations": "Heceta Beacon,Shepherd Base,Mullane Point"
+ },
+ {
+ "name": "Col 285 Sector ID-I c10-26",
+ "pos_x": -142.125,
+ "pos_y": 40.5625,
+ "pos_z": 82.625,
+ "stations": "Salam Horizons"
+ },
+ {
+ "name": "Col 285 Sector ID-I c10-3",
+ "pos_x": -119.1875,
+ "pos_y": 41.125,
+ "pos_z": 68.15625,
+ "stations": "Fisher's Inheritance"
+ },
+ {
+ "name": "Col 285 Sector ID-I c10-6",
+ "pos_x": -113.21875,
+ "pos_y": 16,
+ "pos_z": 88.4375,
+ "stations": "Dobrovolskiy Point"
+ },
+ {
+ "name": "Col 285 Sector IH-I b11-4",
+ "pos_x": 74.28125,
+ "pos_y": 83.34375,
+ "pos_z": -107.0625,
+ "stations": "McDonald Hub"
+ },
+ {
+ "name": "Col 285 Sector IL-D b13-1",
+ "pos_x": 146.59375,
+ "pos_y": -62.875,
+ "pos_z": -82.8125,
+ "stations": "Eilenberg Terminal,Blair Base"
+ },
+ {
+ "name": "Col 285 Sector IR-V d2-36",
+ "pos_x": 108.15625,
+ "pos_y": 62.125,
+ "pos_z": -124.03125,
+ "stations": "Fuchs Survey,Morris Settlement,Nourse Barracks"
+ },
+ {
+ "name": "Col 285 Sector IR-V d2-68",
+ "pos_x": 117.78125,
+ "pos_y": 100.78125,
+ "pos_z": -142.53125,
+ "stations": "Savorgnan de Brazza's Claim"
+ },
+ {
+ "name": "Col 285 Sector IT-W b16-2",
+ "pos_x": -92.0625,
+ "pos_y": -14.28125,
+ "pos_z": -4.625,
+ "stations": "The Heart of Orion"
+ },
+ {
+ "name": "Col 285 Sector IX-T d3-43",
+ "pos_x": -174.75,
+ "pos_y": 73.375,
+ "pos_z": -92.84375,
+ "stations": "Canonn Institute,Kagan Vision,Phillifent Point"
+ },
+ {
+ "name": "Col 285 Sector IX-T d3-81",
+ "pos_x": -165.9375,
+ "pos_y": 68.34375,
+ "pos_z": -36.875,
+ "stations": "Robinson Reach,Mitchell Vision"
+ },
+ {
+ "name": "Col 285 Sector JC-U d3-70",
+ "pos_x": 16.25,
+ "pos_y": 152.65625,
+ "pos_z": -77.21875,
+ "stations": "Gresh Plant,Fernandez Landing"
+ },
+ {
+ "name": "Col 285 Sector JC-U d3-71",
+ "pos_x": 43.4375,
+ "pos_y": 144.75,
+ "pos_z": -103.65625,
+ "stations": "Broglie's Progress,Ochoa Installation"
+ },
+ {
+ "name": "Col 285 Sector JH-I b11-4",
+ "pos_x": 79.09375,
+ "pos_y": 81.84375,
+ "pos_z": -111.15625,
+ "stations": "Burroughs Horizons"
+ },
+ {
+ "name": "Col 285 Sector JH-V d2-66",
+ "pos_x": -142.71875,
+ "pos_y": -37.90625,
+ "pos_z": -112.46875,
+ "stations": "Shatner Enterprise,Shosuke's Inheritance"
+ },
+ {
+ "name": "Col 285 Sector JI-O b21-6",
+ "pos_x": 122.0625,
+ "pos_y": 29.9375,
+ "pos_z": 102.46875,
+ "stations": "Haarsma Observatory"
+ },
+ {
+ "name": "Col 285 Sector JJ-E b13-4",
+ "pos_x": -162.96875,
+ "pos_y": 40.75,
+ "pos_z": -72.5,
+ "stations": "Huxley Plant"
+ },
+ {
+ "name": "Col 285 Sector JM-L c8-20",
+ "pos_x": 164.03125,
+ "pos_y": -20.625,
+ "pos_z": -17.9375,
+ "stations": "Zubrin Base,Meredith Keep"
+ },
+ {
+ "name": "Col 285 Sector JM-L c8-24",
+ "pos_x": 148.15625,
+ "pos_y": -14.5625,
+ "pos_z": -11.09375,
+ "stations": "Slonczewski Penal colony,Bulgarin Installation"
+ },
+ {
+ "name": "Col 285 Sector JO-D b14-3",
+ "pos_x": -37.375,
+ "pos_y": 150.59375,
+ "pos_z": -60.5625,
+ "stations": "Khrenov Base"
+ },
+ {
+ "name": "Col 285 Sector JO-N b21-6",
+ "pos_x": -43.40625,
+ "pos_y": -53.34375,
+ "pos_z": 98.1875,
+ "stations": "Clark Terminal,Crown Relay"
+ },
+ {
+ "name": "Col 285 Sector JR-A b15-3",
+ "pos_x": 162.90625,
+ "pos_y": 22.09375,
+ "pos_z": -35.34375,
+ "stations": "Nagata Refinery"
+ },
+ {
+ "name": "Col 285 Sector JY-H c10-27",
+ "pos_x": -178.65625,
+ "pos_y": -0.34375,
+ "pos_z": 58.78125,
+ "stations": "Elder Holdings"
+ },
+ {
+ "name": "Col 285 Sector JY-H c10-34",
+ "pos_x": -156.40625,
+ "pos_y": -21.875,
+ "pos_z": 64.9375,
+ "stations": "Clair Plant,Turzillo Horizons"
+ },
+ {
+ "name": "Col 285 Sector KF-S b19-3",
+ "pos_x": -125.03125,
+ "pos_y": 64.1875,
+ "pos_z": 60.65625,
+ "stations": "Tucker Laboratory,Appel Hub"
+ },
+ {
+ "name": "Col 285 Sector KH-K a38-3",
+ "pos_x": -141.78125,
+ "pos_y": -34,
+ "pos_z": 62.6875,
+ "stations": "Baydukov Survey"
+ },
+ {
+ "name": "Col 285 Sector KJ-E b13-5",
+ "pos_x": -137.6875,
+ "pos_y": 53.1875,
+ "pos_z": -78.90625,
+ "stations": "Lobachevsky Landing,Kurland Survey"
+ },
+ {
+ "name": "Col 285 Sector KM-V d2-106",
+ "pos_x": 121.5625,
+ "pos_y": 34.625,
+ "pos_z": -128.71875,
+ "stations": "Joule Installation"
+ },
+ {
+ "name": "Col 285 Sector KM-V d2-69",
+ "pos_x": 145.125,
+ "pos_y": 31.625,
+ "pos_z": -115.5,
+ "stations": "Bulgarin Point"
+ },
+ {
+ "name": "Col 285 Sector KM-V d2-83",
+ "pos_x": 128.28125,
+ "pos_y": 47.25,
+ "pos_z": -139.125,
+ "stations": "Henderson Hub"
+ },
+ {
+ "name": "Col 285 Sector KN-I c10-11",
+ "pos_x": 122.9375,
+ "pos_y": 129.875,
+ "pos_z": 55.4375,
+ "stations": "Budrys' Folly"
+ },
+ {
+ "name": "Col 285 Sector KN-I c10-14",
+ "pos_x": 116.34375,
+ "pos_y": 133.78125,
+ "pos_z": 75.5625,
+ "stations": "Gwynn Terminal"
+ },
+ {
+ "name": "Col 285 Sector KS-T d3-124",
+ "pos_x": -168.21875,
+ "pos_y": 40.28125,
+ "pos_z": -100.375,
+ "stations": "Baker Depot"
+ },
+ {
+ "name": "Col 285 Sector KS-T d3-78",
+ "pos_x": -174.0625,
+ "pos_y": 7.96875,
+ "pos_z": -101.71875,
+ "stations": "McCaffrey's Inheritance,Anthony Depot"
+ },
+ {
+ "name": "Col 285 Sector KS-T d3-82",
+ "pos_x": -169.21875,
+ "pos_y": 35.3125,
+ "pos_z": -71.84375,
+ "stations": "Alpers Prospect,Ron Hubbard Prospect"
+ },
+ {
+ "name": "Col 285 Sector KS-T d3-88",
+ "pos_x": -173.40625,
+ "pos_y": 11.78125,
+ "pos_z": -62.15625,
+ "stations": "Pinzon Port"
+ },
+ {
+ "name": "Col 285 Sector KS-T d3-92",
+ "pos_x": -207.71875,
+ "pos_y": 49.53125,
+ "pos_z": -48.375,
+ "stations": "Ellison Legacy"
+ },
+ {
+ "name": "Col 285 Sector KT-F b12-0",
+ "pos_x": -140.71875,
+ "pos_y": -2.4375,
+ "pos_z": -103.4375,
+ "stations": "Ryman Beacon"
+ },
+ {
+ "name": "Col 285 Sector KT-M b22-4",
+ "pos_x": 100.84375,
+ "pos_y": 38.59375,
+ "pos_z": 129.625,
+ "stations": "Womack Terminal,Tavares Base"
+ },
+ {
+ "name": "Col 285 Sector KX-T d3-88",
+ "pos_x": 12.1875,
+ "pos_y": 114.09375,
+ "pos_z": -96.125,
+ "stations": "Vogel Vision"
+ },
+ {
+ "name": "Col 285 Sector KY-H c10-20",
+ "pos_x": -138.78125,
+ "pos_y": -10.53125,
+ "pos_z": 84.375,
+ "stations": "Bering Beacon"
+ },
+ {
+ "name": "Col 285 Sector KY-H c10-26",
+ "pos_x": -118.09375,
+ "pos_y": 10.125,
+ "pos_z": 62.09375,
+ "stations": "Brust Depot"
+ },
+ {
+ "name": "Col 285 Sector KY-H c10-29",
+ "pos_x": -139.75,
+ "pos_y": -23.3125,
+ "pos_z": 68.90625,
+ "stations": "Babbage Refinery,Eddington Vision"
+ },
+ {
+ "name": "Col 285 Sector KY-H c10-31",
+ "pos_x": -141.75,
+ "pos_y": -20.28125,
+ "pos_z": 71.625,
+ "stations": "Chalker Arsenal,Bokeili Mine,Ford Beacon"
+ },
+ {
+ "name": "Col 285 Sector KZ-C b14-1",
+ "pos_x": -133.21875,
+ "pos_y": 79.1875,
+ "pos_z": -64.84375,
+ "stations": "Shaikh Landing,Bixby Installation"
+ },
+ {
+ "name": "Col 285 Sector LD-F b13-2",
+ "pos_x": 53.59375,
+ "pos_y": 125.25,
+ "pos_z": -75.3125,
+ "stations": "Anthony de la Roche Reach"
+ },
+ {
+ "name": "Col 285 Sector LD-O b21-7",
+ "pos_x": 125.5625,
+ "pos_y": 9.71875,
+ "pos_z": 105.21875,
+ "stations": "Yaping Terminal,Burton Barracks"
+ },
+ {
+ "name": "Col 285 Sector LE-L b23-4",
+ "pos_x": 82.65625,
+ "pos_y": 59.46875,
+ "pos_z": 151.875,
+ "stations": "Phillifent Depot"
+ },
+ {
+ "name": "Col 285 Sector LF-J b24-6",
+ "pos_x": -32.75,
+ "pos_y": 45.125,
+ "pos_z": 161.53125,
+ "stations": "Euthymenes Installation"
+ },
+ {
+ "name": "Col 285 Sector LH-L c8-9",
+ "pos_x": 162.46875,
+ "pos_y": -32.5,
+ "pos_z": -9.4375,
+ "stations": "H. G. Wells Beacon,Baxter Base"
+ },
+ {
+ "name": "Col 285 Sector LK-K b23-1",
+ "pos_x": -72.5625,
+ "pos_y": -5.1875,
+ "pos_z": 144.40625,
+ "stations": "Kier Landing"
+ },
+ {
+ "name": "Col 285 Sector LK-T b18-1",
+ "pos_x": -150.25,
+ "pos_y": -1.5625,
+ "pos_z": 48.28125,
+ "stations": "Lewitt Installation"
+ },
+ {
+ "name": "Col 285 Sector LO-G c11-14",
+ "pos_x": -100.25,
+ "pos_y": 64.09375,
+ "pos_z": 116.875,
+ "stations": "Tem Hub"
+ },
+ {
+ "name": "Col 285 Sector LO-G c11-15",
+ "pos_x": -86.96875,
+ "pos_y": 67.125,
+ "pos_z": 107,
+ "stations": "Hendel Observatory,Clairaut Survey"
+ },
+ {
+ "name": "Col 285 Sector LS-G b12-1",
+ "pos_x": 91.25,
+ "pos_y": 104.21875,
+ "pos_z": -95.625,
+ "stations": "Kozlov Mines,Lenoir's Progress"
+ },
+ {
+ "name": "Col 285 Sector LS-K c8-16",
+ "pos_x": -78.46875,
+ "pos_y": -182.15625,
+ "pos_z": 7.21875,
+ "stations": "Mitropoulos Terminal"
+ },
+ {
+ "name": "Col 285 Sector LS-K c8-8",
+ "pos_x": -82.78125,
+ "pos_y": -167.15625,
+ "pos_z": -24.75,
+ "stations": "Arber Base"
+ },
+ {
+ "name": "Col 285 Sector LT-H c10-5",
+ "pos_x": -166.28125,
+ "pos_y": -43.6875,
+ "pos_z": 83.46875,
+ "stations": "Tennyson d'Eyncourt Refinery"
+ },
+ {
+ "name": "Col 285 Sector LX-T d3-74",
+ "pos_x": 94.96875,
+ "pos_y": 83.375,
+ "pos_z": -70.5,
+ "stations": "Searfoss Vision,Doctorow Relay,West Base"
+ },
+ {
+ "name": "Col 285 Sector LX-Y b15-3",
+ "pos_x": 128.75,
+ "pos_y": 27.625,
+ "pos_z": -16.6875,
+ "stations": "Eskridge Barracks,Jenkinson Reach"
+ },
+ {
+ "name": "Col 285 Sector MA-J b24-3",
+ "pos_x": -57.125,
+ "pos_y": 33.34375,
+ "pos_z": 158.0625,
+ "stations": "Aldiss Terminal"
+ },
+ {
+ "name": "Col 285 Sector MA-R b20-2",
+ "pos_x": -49.15625,
+ "pos_y": 123.59375,
+ "pos_z": 77.34375,
+ "stations": "Lindbohm Port"
+ },
+ {
+ "name": "Col 285 Sector MA-R b20-3",
+ "pos_x": -53.03125,
+ "pos_y": 123.78125,
+ "pos_z": 76.90625,
+ "stations": "Godwin Base,Fontenay Barracks"
+ },
+ {
+ "name": "Col 285 Sector MD-S d4-42",
+ "pos_x": -160.1875,
+ "pos_y": 94.65625,
+ "pos_z": 9.21875,
+ "stations": "Brust Forum"
+ },
+ {
+ "name": "Col 285 Sector MD-S d4-45",
+ "pos_x": -145.8125,
+ "pos_y": 96.71875,
+ "pos_z": 50.34375,
+ "stations": "Schachner Beacon"
+ },
+ {
+ "name": "Col 285 Sector MF-R b20-1",
+ "pos_x": -23.03125,
+ "pos_y": 142.40625,
+ "pos_z": 83.625,
+ "stations": "Verrazzano Survey"
+ },
+ {
+ "name": "Col 285 Sector MJ-G c11-1",
+ "pos_x": -120.375,
+ "pos_y": 26.75,
+ "pos_z": 112.15625,
+ "stations": "Turzillo Survey,Ing's Inheritance"
+ },
+ {
+ "name": "Col 285 Sector MJ-G c11-25",
+ "pos_x": -124.9375,
+ "pos_y": 44.09375,
+ "pos_z": 132.375,
+ "stations": "Paulmier de Gonneville Escape"
+ },
+ {
+ "name": "Col 285 Sector MP-S b19-3",
+ "pos_x": -8.84375,
+ "pos_y": 97.46875,
+ "pos_z": 72.375,
+ "stations": "Fisk's Claim"
+ },
+ {
+ "name": "Col 285 Sector MT-G c11-5",
+ "pos_x": 22.09375,
+ "pos_y": 99.75,
+ "pos_z": 133.84375,
+ "stations": "Helmholtz Market"
+ },
+ {
+ "name": "Col 285 Sector MX-T d3-65",
+ "pos_x": 107.21875,
+ "pos_y": 98.78125,
+ "pos_z": -81.78125,
+ "stations": "Margulies Depot,Crowley Depot"
+ },
+ {
+ "name": "Col 285 Sector MX-T d3-86",
+ "pos_x": 110.53125,
+ "pos_y": 131.875,
+ "pos_z": -86.09375,
+ "stations": "Stableford Landing"
+ },
+ {
+ "name": "Col 285 Sector MX-T d3-93",
+ "pos_x": 151.96875,
+ "pos_y": 120.59375,
+ "pos_z": -61.625,
+ "stations": "Tsunenaga Reach"
+ },
+ {
+ "name": "Col 285 Sector NA-S b19-5",
+ "pos_x": -114.4375,
+ "pos_y": 49.78125,
+ "pos_z": 71.75,
+ "stations": "Cook Base,Tenn Camp"
+ },
+ {
+ "name": "Col 285 Sector ND-S d4-70",
+ "pos_x": -140.125,
+ "pos_y": 71.03125,
+ "pos_z": 38.21875,
+ "stations": "Herjulfsson Vision,Hutton Prospect"
+ },
+ {
+ "name": "Col 285 Sector NH-A b15-4",
+ "pos_x": 167.53125,
+ "pos_y": -8.28125,
+ "pos_z": -39.625,
+ "stations": "Boulle Palace"
+ },
+ {
+ "name": "Col 285 Sector NI-R c5-8",
+ "pos_x": -17.59375,
+ "pos_y": 127.96875,
+ "pos_z": -112.75,
+ "stations": "Ellison Vision"
+ },
+ {
+ "name": "Col 285 Sector NI-S d4-69",
+ "pos_x": 44.9375,
+ "pos_y": 152.125,
+ "pos_z": 35.21875,
+ "stations": "Reynolds Hub"
+ },
+ {
+ "name": "Col 285 Sector NN-Z a43-0",
+ "pos_x": -113.78125,
+ "pos_y": -44.5625,
+ "pos_z": 115.21875,
+ "stations": "Nicollet Mine"
+ },
+ {
+ "name": "Col 285 Sector NO-M b22-1",
+ "pos_x": 124.5,
+ "pos_y": 25.15625,
+ "pos_z": 123,
+ "stations": "Barjavel Plant"
+ },
+ {
+ "name": "Col 285 Sector NS-J c9-5",
+ "pos_x": 170.59375,
+ "pos_y": -6.09375,
+ "pos_z": 47.875,
+ "stations": "Kazantsev Prospect"
+ },
+ {
+ "name": "Col 285 Sector NU-L b22-4",
+ "pos_x": -33.90625,
+ "pos_y": -65,
+ "pos_z": 115.71875,
+ "stations": "Wilson Landing,Birkhoff Settlement"
+ },
+ {
+ "name": "Col 285 Sector NU-L b22-5",
+ "pos_x": -28.15625,
+ "pos_y": -46.75,
+ "pos_z": 117.15625,
+ "stations": "Barton Settlement,Schachner Settlement"
+ },
+ {
+ "name": "Col 285 Sector OI-R c5-6",
+ "pos_x": 52.375,
+ "pos_y": 113.15625,
+ "pos_z": -114.125,
+ "stations": "Brunel Terminal"
+ },
+ {
+ "name": "Col 285 Sector ON-G b12-2",
+ "pos_x": 114.71875,
+ "pos_y": 80.625,
+ "pos_z": -88.6875,
+ "stations": "Gotlieb Observatory"
+ },
+ {
+ "name": "Col 285 Sector OO-P c6-4",
+ "pos_x": -115.125,
+ "pos_y": 114.71875,
+ "pos_z": -72.34375,
+ "stations": "Lunan Base"
+ },
+ {
+ "name": "Col 285 Sector OS-T d3-104",
+ "pos_x": 163.15625,
+ "pos_y": 19.40625,
+ "pos_z": -29.3125,
+ "stations": "McKay's Progress"
+ },
+ {
+ "name": "Col 285 Sector OS-T d3-146",
+ "pos_x": 129.8125,
+ "pos_y": 9.03125,
+ "pos_z": -86.21875,
+ "stations": "Judson Settlement"
+ },
+ {
+ "name": "Col 285 Sector OS-T d3-64",
+ "pos_x": 168.78125,
+ "pos_y": 39.90625,
+ "pos_z": -45.65625,
+ "stations": "Dalmas Port"
+ },
+ {
+ "name": "Col 285 Sector OS-T d3-70",
+ "pos_x": 142.125,
+ "pos_y": -22.40625,
+ "pos_z": -104.96875,
+ "stations": "Abbott Settlement"
+ },
+ {
+ "name": "Col 285 Sector OS-T d3-96",
+ "pos_x": 149.03125,
+ "pos_y": 20.375,
+ "pos_z": -103.5625,
+ "stations": "Stephenson Gateway"
+ },
+ {
+ "name": "Col 285 Sector OS-T d3-99",
+ "pos_x": 174.65625,
+ "pos_y": -17.96875,
+ "pos_z": -98.59375,
+ "stations": "Ledyard Port"
+ },
+ {
+ "name": "Col 285 Sector OT-G c11-12",
+ "pos_x": 109.4375,
+ "pos_y": 98.4375,
+ "pos_z": 127.5,
+ "stations": "Teng Depot"
+ },
+ {
+ "name": "Col 285 Sector OT-G c11-3",
+ "pos_x": 127.625,
+ "pos_y": 110.96875,
+ "pos_z": 95.09375,
+ "stations": "Cauchy Observatory,Naddoddur Survey"
+ },
+ {
+ "name": "Col 285 Sector OT-G c11-6",
+ "pos_x": 127.25,
+ "pos_y": 108.21875,
+ "pos_z": 100.15625,
+ "stations": "Riemann Vision,Mourelle Landing,Birmingham Landing"
+ },
+ {
+ "name": "Col 285 Sector OT-P c6-7",
+ "pos_x": -33.375,
+ "pos_y": 153.34375,
+ "pos_z": -96.40625,
+ "stations": "Paez's Inheritance"
+ },
+ {
+ "name": "Col 285 Sector OV-I b24-4",
+ "pos_x": -51.96875,
+ "pos_y": 4.6875,
+ "pos_z": 162.125,
+ "stations": "Pierce Settlement"
+ },
+ {
+ "name": "Col 285 Sector OY-R d4-104",
+ "pos_x": -210.09375,
+ "pos_y": 32.90625,
+ "pos_z": -8.3125,
+ "stations": "Thurston Enterprise,Greenland Enterprise"
+ },
+ {
+ "name": "Col 285 Sector OY-R d4-128",
+ "pos_x": -145.4375,
+ "pos_y": 7.125,
+ "pos_z": -12.375,
+ "stations": "Lopez de Legazpi Point,Tolstoi Installation,Kelly Terminal"
+ },
+ {
+ "name": "Col 285 Sector OY-R d4-62",
+ "pos_x": -221.65625,
+ "pos_y": 19.28125,
+ "pos_z": -11.125,
+ "stations": "Eilenberg Prospect"
+ },
+ {
+ "name": "Col 285 Sector OY-R d4-76",
+ "pos_x": -160.34375,
+ "pos_y": 44.71875,
+ "pos_z": 11.5,
+ "stations": "Moskowitz Refinery,Sladek Beacon,McHugh Landing"
+ },
+ {
+ "name": "Col 285 Sector OZ-B b15-3",
+ "pos_x": 29.59375,
+ "pos_y": 155.625,
+ "pos_z": -40.40625,
+ "stations": "Pangborn Town"
+ },
+ {
+ "name": "Col 285 Sector PE-G c11-20",
+ "pos_x": -86.96875,
+ "pos_y": -16.3125,
+ "pos_z": 110.5,
+ "stations": "Leichhardt Beacon,Garden Barracks"
+ },
+ {
+ "name": "Col 285 Sector PE-G c11-6",
+ "pos_x": -96.5,
+ "pos_y": -6.6875,
+ "pos_z": 104.15625,
+ "stations": "Ibold Installation,Lewis Silo"
+ },
+ {
+ "name": "Col 285 Sector PE-G c11-8",
+ "pos_x": -99.34375,
+ "pos_y": -6.9375,
+ "pos_z": 108.6875,
+ "stations": "Springer's Inheritance,Fu Enterprise"
+ },
+ {
+ "name": "Col 285 Sector PI-R c5-6",
+ "pos_x": 75.90625,
+ "pos_y": 127.375,
+ "pos_z": -123.9375,
+ "stations": "Peano Palace"
+ },
+ {
+ "name": "Col 285 Sector PI-R c5-9",
+ "pos_x": 81,
+ "pos_y": 134.96875,
+ "pos_z": -106.25,
+ "stations": "Gaiman Oasis"
+ },
+ {
+ "name": "Col 285 Sector PJ-M b22-6",
+ "pos_x": 127,
+ "pos_y": 6.84375,
+ "pos_z": 126.6875,
+ "stations": "Przhevalsky Works"
+ },
+ {
+ "name": "Col 285 Sector PN-G b12-4",
+ "pos_x": 117.5625,
+ "pos_y": 77.3125,
+ "pos_z": -87.3125,
+ "stations": "Franklin Vision"
+ },
+ {
+ "name": "Col 285 Sector PO-G c11-22",
+ "pos_x": 89.5625,
+ "pos_y": 94.75,
+ "pos_z": 134.46875,
+ "stations": "Gerlache Prospect"
+ },
+ {
+ "name": "Col 285 Sector PO-G c11-3",
+ "pos_x": 63.5,
+ "pos_y": 61.53125,
+ "pos_z": 133.9375,
+ "stations": "Harrison Base,Klein Arsenal,Brillant Vision"
+ },
+ {
+ "name": "Col 285 Sector PQ-R b19-6",
+ "pos_x": -160.15625,
+ "pos_y": 13.03125,
+ "pos_z": 58.5,
+ "stations": "Cadamosto Barracks"
+ },
+ {
+ "name": "Col 285 Sector PU-E c12-20",
+ "pos_x": -93.0625,
+ "pos_y": 58.0625,
+ "pos_z": 145.5625,
+ "stations": "Clarke's Folly"
+ },
+ {
+ "name": "Col 285 Sector PV-Z b15-1",
+ "pos_x": -119.21875,
+ "pos_y": 134.8125,
+ "pos_z": -21.21875,
+ "stations": "Evans Settlement,Williams Keep"
+ },
+ {
+ "name": "Col 285 Sector PY-I c9-11",
+ "pos_x": -83.34375,
+ "pos_y": -159.8125,
+ "pos_z": 41.125,
+ "stations": "Schiaparelli Prospect,MacLeod Terminal"
+ },
+ {
+ "name": "Col 285 Sector PY-I c9-13",
+ "pos_x": -74.15625,
+ "pos_y": -176.9375,
+ "pos_z": 30.3125,
+ "stations": "Kozin Reach,Pippin Enterprise"
+ },
+ {
+ "name": "Col 285 Sector PY-R d4-100",
+ "pos_x": -127.0625,
+ "pos_y": 49.1875,
+ "pos_z": 46.65625,
+ "stations": "Sekowski Landing"
+ },
+ {
+ "name": "Col 285 Sector PY-R d4-140",
+ "pos_x": -136.53125,
+ "pos_y": 28.59375,
+ "pos_z": 40.0625,
+ "stations": "Nobel's Folly,Galiano Point"
+ },
+ {
+ "name": "Col 285 Sector PY-R d4-75",
+ "pos_x": -135.3125,
+ "pos_y": -10.125,
+ "pos_z": 52.15625,
+ "stations": "Linenger Base"
+ },
+ {
+ "name": "Col 285 Sector PY-R d4-81",
+ "pos_x": -134.53125,
+ "pos_y": -11.71875,
+ "pos_z": 24.65625,
+ "stations": "Cassidy Terminal,Bamford Settlement,Santos Mine"
+ },
+ {
+ "name": "Col 285 Sector QE-M b22-3",
+ "pos_x": 102.34375,
+ "pos_y": -20.625,
+ "pos_z": 121.25,
+ "stations": "Przhevalsky Terminal"
+ },
+ {
+ "name": "Col 285 Sector QJ-H c10-23",
+ "pos_x": -129.21875,
+ "pos_y": -113.65625,
+ "pos_z": 90.5625,
+ "stations": "Goldstein Settlement"
+ },
+ {
+ "name": "Col 285 Sector QJ-P c6-15",
+ "pos_x": -128.90625,
+ "pos_y": 91.625,
+ "pos_z": -68.34375,
+ "stations": "Budrys Vision"
+ },
+ {
+ "name": "Col 285 Sector QJ-P c6-16",
+ "pos_x": -133.25,
+ "pos_y": 93.1875,
+ "pos_z": -69.59375,
+ "stations": "Gordon's Inheritance"
+ },
+ {
+ "name": "Col 285 Sector QJ-P c6-18",
+ "pos_x": -136.25,
+ "pos_y": 78.0625,
+ "pos_z": -90.125,
+ "stations": "Christopher Landing"
+ },
+ {
+ "name": "Col 285 Sector QJ-Q d5-47",
+ "pos_x": -191.9375,
+ "pos_y": 76.90625,
+ "pos_z": 83.5625,
+ "stations": "Baffin Horizons"
+ },
+ {
+ "name": "Col 285 Sector QJ-Q d5-50",
+ "pos_x": -146.59375,
+ "pos_y": 102.84375,
+ "pos_z": 67.375,
+ "stations": "Dias Market"
+ },
+ {
+ "name": "Col 285 Sector QN-T d3-77",
+ "pos_x": 163.03125,
+ "pos_y": -38.125,
+ "pos_z": -44.53125,
+ "stations": "Herndon Plant,Gunn Horizons"
+ },
+ {
+ "name": "Col 285 Sector QO-N b21-5",
+ "pos_x": 96.4375,
+ "pos_y": -58.375,
+ "pos_z": 100.46875,
+ "stations": "Apollo's Vengeance"
+ },
+ {
+ "name": "Col 285 Sector QQ-I b24-3",
+ "pos_x": -62.71875,
+ "pos_y": -20.09375,
+ "pos_z": 158.34375,
+ "stations": "Norman Vista"
+ },
+ {
+ "name": "Col 285 Sector QQ-R b19-1",
+ "pos_x": -139.4375,
+ "pos_y": -2,
+ "pos_z": 55.71875,
+ "stations": "Gaspar de Portola's Exile,Bester Base,Artin Base"
+ },
+ {
+ "name": "Col 285 Sector QT-E b13-4",
+ "pos_x": 72.90625,
+ "pos_y": 75.6875,
+ "pos_z": -84.375,
+ "stations": "The Redeemer"
+ },
+ {
+ "name": "Col 285 Sector QT-P c6-13",
+ "pos_x": 31.875,
+ "pos_y": 148.78125,
+ "pos_z": -101.25,
+ "stations": "Kingsbury Exchange"
+ },
+ {
+ "name": "Col 285 Sector QT-P c6-7",
+ "pos_x": 43.71875,
+ "pos_y": 147.78125,
+ "pos_z": -93.1875,
+ "stations": "Barbaro Vista"
+ },
+ {
+ "name": "Col 285 Sector QT-Q c5-10",
+ "pos_x": -134.90625,
+ "pos_y": 6.65625,
+ "pos_z": -117.34375,
+ "stations": "Mitchell Palace"
+ },
+ {
+ "name": "Col 285 Sector QU-B b15-1",
+ "pos_x": 33.71875,
+ "pos_y": 150.4375,
+ "pos_z": -41.53125,
+ "stations": "Cunningham Base"
+ },
+ {
+ "name": "Col 285 Sector QY-X a44-4",
+ "pos_x": -95.4375,
+ "pos_y": -27.53125,
+ "pos_z": 132.5625,
+ "stations": "Vardeman Horizons"
+ },
+ {
+ "name": "Col 285 Sector QZ-F c11-10",
+ "pos_x": -117.65625,
+ "pos_y": -40.15625,
+ "pos_z": 127.3125,
+ "stations": "Weil Legacy"
+ },
+ {
+ "name": "Col 285 Sector QZ-F c11-2",
+ "pos_x": -116.1875,
+ "pos_y": -30.5625,
+ "pos_z": 116.9375,
+ "stations": "Henson Base"
+ },
+ {
+ "name": "Col 285 Sector QZ-F c11-8",
+ "pos_x": -141.1875,
+ "pos_y": -30.8125,
+ "pos_z": 117.40625,
+ "stations": "Atiyah Terminal"
+ },
+ {
+ "name": "Col 285 Sector RE-P c6-5",
+ "pos_x": -178.46875,
+ "pos_y": 31.28125,
+ "pos_z": -72.75,
+ "stations": "Sucharitkul Lab,Czerneda Oasis"
+ },
+ {
+ "name": "Col 285 Sector RE-P c6-8",
+ "pos_x": -184.15625,
+ "pos_y": 25,
+ "pos_z": -78.4375,
+ "stations": "Back Horizons,Desargues' Folly,Barth Base"
+ },
+ {
+ "name": "Col 285 Sector RF-C b14-1",
+ "pos_x": -157.40625,
+ "pos_y": -3.1875,
+ "pos_z": -62.125,
+ "stations": "Clark Point"
+ },
+ {
+ "name": "Col 285 Sector RJ-Q d5-81",
+ "pos_x": -65.34375,
+ "pos_y": 103.625,
+ "pos_z": 113.875,
+ "stations": "Navigator Prospect"
+ },
+ {
+ "name": "Col 285 Sector RJ-Q d5-90",
+ "pos_x": -75.125,
+ "pos_y": 121,
+ "pos_z": 92.125,
+ "stations": "Clarke Terminal"
+ },
+ {
+ "name": "Col 285 Sector RL-R b19-5",
+ "pos_x": -146.5,
+ "pos_y": -7.8125,
+ "pos_z": 63.375,
+ "stations": "Antonio de Andrade Enterprise,Clark Beacon,Gilliland Vision"
+ },
+ {
+ "name": "Col 285 Sector RL-R b19-6",
+ "pos_x": -161.96875,
+ "pos_y": -8.0625,
+ "pos_z": 69.1875,
+ "stations": "Godwin's Inheritance"
+ },
+ {
+ "name": "Col 285 Sector RL-Z b15-0",
+ "pos_x": -162.375,
+ "pos_y": 77.53125,
+ "pos_z": -23.9375,
+ "stations": "Bohr Reach"
+ },
+ {
+ "name": "Col 285 Sector RO-Q d5-54",
+ "pos_x": 32.8125,
+ "pos_y": 158.8125,
+ "pos_z": 85.8125,
+ "stations": "Chilton Settlement"
+ },
+ {
+ "name": "Col 285 Sector RP-E c12-19",
+ "pos_x": -70.34375,
+ "pos_y": 49.09375,
+ "pos_z": 159.0625,
+ "stations": "Pelt Keep"
+ },
+ {
+ "name": "Col 285 Sector RP-E c12-5",
+ "pos_x": -103.5,
+ "pos_y": 54.5625,
+ "pos_z": 141.78125,
+ "stations": "Dozois Works"
+ },
+ {
+ "name": "Col 285 Sector RP-K b23-6",
+ "pos_x": 94.4375,
+ "pos_y": 11.0625,
+ "pos_z": 147.84375,
+ "stations": "Stiegler Point"
+ },
+ {
+ "name": "Col 285 Sector RQ-I b24-8",
+ "pos_x": -35.28125,
+ "pos_y": -23.96875,
+ "pos_z": 173.375,
+ "stations": "Morgan Landing"
+ },
+ {
+ "name": "Col 285 Sector RT-P c6-7",
+ "pos_x": 57.46875,
+ "pos_y": 164.65625,
+ "pos_z": -66.59375,
+ "stations": "Virts Palace"
+ },
+ {
+ "name": "Col 285 Sector RU-E c12-6",
+ "pos_x": -12.4375,
+ "pos_y": 81.75,
+ "pos_z": 153.6875,
+ "stations": "Forest Terminal"
+ },
+ {
+ "name": "Col 285 Sector RU-E c12-8",
+ "pos_x": -14.625,
+ "pos_y": 85.375,
+ "pos_z": 145.625,
+ "stations": "Lenoir Depot,Walters Depot"
+ },
+ {
+ "name": "Col 285 Sector RV-Q b20-3",
+ "pos_x": -2.0625,
+ "pos_y": 99.9375,
+ "pos_z": 90.15625,
+ "stations": "Bendell Landing,Werber's Folly,Leichhardt Works"
+ },
+ {
+ "name": "Col 285 Sector RY-H c10-20",
+ "pos_x": 167.78125,
+ "pos_y": -8.34375,
+ "pos_z": 60.53125,
+ "stations": "Guest Enterprise"
+ },
+ {
+ "name": "Col 285 Sector RY-H c10-24",
+ "pos_x": 146.46875,
+ "pos_y": -8.875,
+ "pos_z": 87.28125,
+ "stations": "Bean Enterprise"
+ },
+ {
+ "name": "Col 285 Sector RY-H c10-36",
+ "pos_x": 174.375,
+ "pos_y": -12.5,
+ "pos_z": 82.25,
+ "stations": "Huxley Installation,Ellison's Progress"
+ },
+ {
+ "name": "Col 285 Sector RZ-F c11-19",
+ "pos_x": -91.90625,
+ "pos_y": -56.40625,
+ "pos_z": 118.4375,
+ "stations": "Francisco de Eliza Depot,Diesel Depot"
+ },
+ {
+ "name": "Col 285 Sector SD-R c5-11",
+ "pos_x": 131.75,
+ "pos_y": 89.3125,
+ "pos_z": -122.8125,
+ "stations": "Williams' Pride"
+ },
+ {
+ "name": "Col 285 Sector SD-R c5-14",
+ "pos_x": 95.40625,
+ "pos_y": 80.71875,
+ "pos_z": -132.375,
+ "stations": "Smith Lab"
+ },
+ {
+ "name": "Col 285 Sector SD-R c5-15",
+ "pos_x": 131.125,
+ "pos_y": 80.28125,
+ "pos_z": -107.875,
+ "stations": "Rayhan al-Biruni Depot"
+ },
+ {
+ "name": "Col 285 Sector SE-D b14-0",
+ "pos_x": 58.75,
+ "pos_y": 99.21875,
+ "pos_z": -59.75,
+ "stations": "Ingstad Silo,Williams Beacon"
+ },
+ {
+ "name": "Col 285 Sector SE-P c6-19",
+ "pos_x": -132.375,
+ "pos_y": 39.9375,
+ "pos_z": -93.875,
+ "stations": "Lopez de Villalobos Prospect"
+ },
+ {
+ "name": "Col 285 Sector SE-Q d5-107",
+ "pos_x": -182.21875,
+ "pos_y": 5.625,
+ "pos_z": 55.84375,
+ "stations": "Green Vista"
+ },
+ {
+ "name": "Col 285 Sector SE-Q d5-108",
+ "pos_x": -172.96875,
+ "pos_y": 14.34375,
+ "pos_z": 59.71875,
+ "stations": "Kagan Keep"
+ },
+ {
+ "name": "Col 285 Sector SE-Q d5-125",
+ "pos_x": -170.5625,
+ "pos_y": -23.59375,
+ "pos_z": 112.0625,
+ "stations": "Steven's Town"
+ },
+ {
+ "name": "Col 285 Sector SE-Q d5-134",
+ "pos_x": -178.96875,
+ "pos_y": 30.84375,
+ "pos_z": 71.875,
+ "stations": "Rasch Depot,Baker Arena"
+ },
+ {
+ "name": "Col 285 Sector SE-Q d5-138",
+ "pos_x": -164.09375,
+ "pos_y": -12.78125,
+ "pos_z": 99.09375,
+ "stations": "Alexandrov Base"
+ },
+ {
+ "name": "Col 285 Sector SE-Q d5-142",
+ "pos_x": -162.25,
+ "pos_y": -12.25,
+ "pos_z": 77.125,
+ "stations": "Ing Prospect,Doyle Enterprise"
+ },
+ {
+ "name": "Col 285 Sector SJ-G c11-13",
+ "pos_x": 96.6875,
+ "pos_y": 31.53125,
+ "pos_z": 129.0625,
+ "stations": "Terry Settlement"
+ },
+ {
+ "name": "Col 285 Sector SJ-G c11-17",
+ "pos_x": 119.1875,
+ "pos_y": 54.03125,
+ "pos_z": 120.5625,
+ "stations": "Miletus Survey"
+ },
+ {
+ "name": "Col 285 Sector SJ-G c11-24",
+ "pos_x": 105.53125,
+ "pos_y": 53.8125,
+ "pos_z": 120.96875,
+ "stations": "Krylov Laboratory"
+ },
+ {
+ "name": "Col 285 Sector SJ-G c11-29",
+ "pos_x": 99.3125,
+ "pos_y": 30.15625,
+ "pos_z": 125.3125,
+ "stations": "Cook Exchange"
+ },
+ {
+ "name": "Col 285 Sector SJ-Q d5-85",
+ "pos_x": -9.40625,
+ "pos_y": 83.75,
+ "pos_z": 124.0625,
+ "stations": "Hogan Enterprise,Levy Installation"
+ },
+ {
+ "name": "Col 285 Sector SL-Z b15-3",
+ "pos_x": -128.21875,
+ "pos_y": 89.4375,
+ "pos_z": -13.25,
+ "stations": "Jones Vision"
+ },
+ {
+ "name": "Col 285 Sector SO-P c6-11",
+ "pos_x": 47.96875,
+ "pos_y": 108.6875,
+ "pos_z": -82.03125,
+ "stations": "Kippax Base,Ryman Base,Cooper Point"
+ },
+ {
+ "name": "Col 285 Sector SP-E c12-19",
+ "pos_x": -32.4375,
+ "pos_y": 39.71875,
+ "pos_z": 173.15625,
+ "stations": "Barreiros Forum"
+ },
+ {
+ "name": "Col 285 Sector SP-E c12-20",
+ "pos_x": -37.625,
+ "pos_y": 33.78125,
+ "pos_z": 174.1875,
+ "stations": "Rotsler Forum"
+ },
+ {
+ "name": "Col 285 Sector SQ-I b24-8",
+ "pos_x": -18.3125,
+ "pos_y": -9,
+ "pos_z": 160.9375,
+ "stations": "Wilson Beacon,Bester Penal colony"
+ },
+ {
+ "name": "Col 285 Sector SU-E c12-12",
+ "pos_x": 46.15625,
+ "pos_y": 73.625,
+ "pos_z": 141.28125,
+ "stations": "Dayuan Vision,Brendan Camp"
+ },
+ {
+ "name": "Col 285 Sector SU-E c12-18",
+ "pos_x": 35.1875,
+ "pos_y": 72.40625,
+ "pos_z": 154.9375,
+ "stations": "Campbell Stop,Carlisle Survey"
+ },
+ {
+ "name": "Col 285 Sector SW-O b21-2",
+ "pos_x": -101.25,
+ "pos_y": 77.03125,
+ "pos_z": 95.59375,
+ "stations": "Leibniz's Progress"
+ },
+ {
+ "name": "Col 285 Sector SZ-F c11-18",
+ "pos_x": -41.09375,
+ "pos_y": -42.34375,
+ "pos_z": 111.96875,
+ "stations": "Hodgson Depot"
+ },
+ {
+ "name": "Col 285 Sector SZ-F c11-4",
+ "pos_x": -38.09375,
+ "pos_y": -55.75,
+ "pos_z": 104.34375,
+ "stations": "Sladek Installation,Pook Horizons"
+ },
+ {
+ "name": "Col 285 Sector SZ-F c11-6",
+ "pos_x": -34.71875,
+ "pos_y": -61.9375,
+ "pos_z": 133.53125,
+ "stations": "Zoline Horizons"
+ },
+ {
+ "name": "Col 285 Sector TD-T d3-70",
+ "pos_x": 45.34375,
+ "pos_y": -224.125,
+ "pos_z": -85.9375,
+ "stations": "Alexander Escape"
+ },
+ {
+ "name": "Col 285 Sector TE-Q d5-104",
+ "pos_x": -138.75,
+ "pos_y": 9.15625,
+ "pos_z": 113.9375,
+ "stations": "Bova Installation,Mackenzie Vision"
+ },
+ {
+ "name": "Col 285 Sector TE-Q d5-96",
+ "pos_x": -133.5,
+ "pos_y": 37.1875,
+ "pos_z": 96.8125,
+ "stations": "Willis Penal colony,Sturt Vision"
+ },
+ {
+ "name": "Col 285 Sector TE-X b15-0",
+ "pos_x": -81.34375,
+ "pos_y": -163.84375,
+ "pos_z": -21.09375,
+ "stations": "Coke Colony"
+ },
+ {
+ "name": "Col 285 Sector TG-P b21-2",
+ "pos_x": 14.1875,
+ "pos_y": 121.96875,
+ "pos_z": 97.125,
+ "stations": "Navigator Plant"
+ },
+ {
+ "name": "Col 285 Sector TG-Y b16-0",
+ "pos_x": -84.09375,
+ "pos_y": 137.25,
+ "pos_z": -4.625,
+ "stations": "Lupoff Landing,Watts Installation"
+ },
+ {
+ "name": "Col 285 Sector TJ-Q d5-101",
+ "pos_x": 81.71875,
+ "pos_y": 121,
+ "pos_z": 119.3125,
+ "stations": "Leiber's Pride"
+ },
+ {
+ "name": "Col 285 Sector TK-E c12-13",
+ "pos_x": -74.78125,
+ "pos_y": -8.28125,
+ "pos_z": 150.6875,
+ "stations": "Kent Installation,al-Khowarizmi Bastion"
+ },
+ {
+ "name": "Col 285 Sector TK-E c12-16",
+ "pos_x": -83,
+ "pos_y": -12.65625,
+ "pos_z": 165.5,
+ "stations": "Howard Beacon,Grimwood Vista"
+ },
+ {
+ "name": "Col 285 Sector TK-E c12-22",
+ "pos_x": -81.125,
+ "pos_y": -11.71875,
+ "pos_z": 174.1875,
+ "stations": "van Vogt Horizons"
+ },
+ {
+ "name": "Col 285 Sector TK-E c12-4",
+ "pos_x": -88.71875,
+ "pos_y": -16.28125,
+ "pos_z": 163.5,
+ "stations": "Ford Holdings"
+ },
+ {
+ "name": "Col 285 Sector TK-E c12-6",
+ "pos_x": -82.53125,
+ "pos_y": -19.1875,
+ "pos_z": 135.75,
+ "stations": "Campbell Refinery"
+ },
+ {
+ "name": "Col 285 Sector TO-R d4-70",
+ "pos_x": -89.46875,
+ "pos_y": -163.5625,
+ "pos_z": 33.59375,
+ "stations": "Charnas Stop"
+ },
+ {
+ "name": "Col 285 Sector TO-R d4-86",
+ "pos_x": -94.03125,
+ "pos_y": -138.03125,
+ "pos_z": 44.125,
+ "stations": "Sutcliffe Holdings"
+ },
+ {
+ "name": "Col 285 Sector TP-N c7-12",
+ "pos_x": -149.6875,
+ "pos_y": 84.9375,
+ "pos_z": -52.28125,
+ "stations": "Zelazny Oasis"
+ },
+ {
+ "name": "Col 285 Sector TP-N c7-8",
+ "pos_x": -162.0625,
+ "pos_y": 69.46875,
+ "pos_z": -61.90625,
+ "stations": "Vancouver's Inheritance"
+ },
+ {
+ "name": "Col 285 Sector TU-E c12-23",
+ "pos_x": 87.96875,
+ "pos_y": 89.75,
+ "pos_z": 154.5625,
+ "stations": "Doyle Beacon,Moorcock Vision"
+ },
+ {
+ "name": "Col 285 Sector TV-J b23-2",
+ "pos_x": -38.9375,
+ "pos_y": -73.375,
+ "pos_z": 151.4375,
+ "stations": "Rasch Forum"
+ },
+ {
+ "name": "Col 285 Sector TW-O b21-0",
+ "pos_x": -81.15625,
+ "pos_y": 75.71875,
+ "pos_z": 99.21875,
+ "stations": "Rawat Depot,Grassmann Survey"
+ },
+ {
+ "name": "Col 285 Sector TY-W b16-6",
+ "pos_x": 158.5625,
+ "pos_y": 1.4375,
+ "pos_z": 0.1875,
+ "stations": "Guest Installation,Wrangel's Folly,Bao Survey"
+ },
+ {
+ "name": "Col 285 Sector TZ-N c7-11",
+ "pos_x": 8.75,
+ "pos_y": 170.75,
+ "pos_z": -42.09375,
+ "stations": "Nikitin Asylum"
+ },
+ {
+ "name": "Col 285 Sector TZ-O c6-22",
+ "pos_x": -157.25,
+ "pos_y": 11.6875,
+ "pos_z": -75.6875,
+ "stations": "Farmer Forum"
+ },
+ {
+ "name": "Col 285 Sector TZ-O c6-23",
+ "pos_x": -174.1875,
+ "pos_y": -8.09375,
+ "pos_z": -76.25,
+ "stations": "Foden Terminal"
+ },
+ {
+ "name": "Col 285 Sector TZ-O c6-27",
+ "pos_x": -166.375,
+ "pos_y": -17.28125,
+ "pos_z": -95.65625,
+ "stations": "Hiraga Terminal"
+ },
+ {
+ "name": "Col 285 Sector UA-K b23-11",
+ "pos_x": 26.625,
+ "pos_y": -58.4375,
+ "pos_z": 149.59375,
+ "stations": "Wilson Installation"
+ },
+ {
+ "name": "Col 285 Sector UD-G b12-2",
+ "pos_x": 147.03125,
+ "pos_y": 37.9375,
+ "pos_z": -97.5,
+ "stations": "Cormack Depot,Terry Stop,Delany Lab"
+ },
+ {
+ "name": "Col 285 Sector UE-G c11-11",
+ "pos_x": 124.03125,
+ "pos_y": -1.6875,
+ "pos_z": 106.46875,
+ "stations": "Wheelock Hub"
+ },
+ {
+ "name": "Col 285 Sector UE-G c11-19",
+ "pos_x": 120.3125,
+ "pos_y": -19.25,
+ "pos_z": 127.34375,
+ "stations": "Pournelle Relay,King Depot"
+ },
+ {
+ "name": "Col 285 Sector UE-G c11-20",
+ "pos_x": 124.0625,
+ "pos_y": -17.78125,
+ "pos_z": 126.40625,
+ "stations": "Lawhead Terminal,Asher Point,Ocampo Landing"
+ },
+ {
+ "name": "Col 285 Sector UE-G c11-27",
+ "pos_x": 120.65625,
+ "pos_y": -7.34375,
+ "pos_z": 126.625,
+ "stations": "MacLean Enterprise"
+ },
+ {
+ "name": "Col 285 Sector UJ-Q d5-104",
+ "pos_x": 135.46875,
+ "pos_y": 107.78125,
+ "pos_z": 83.59375,
+ "stations": "Cayley Prospect"
+ },
+ {
+ "name": "Col 285 Sector UJ-Q d5-111",
+ "pos_x": 119.03125,
+ "pos_y": 110.75,
+ "pos_z": 122.59375,
+ "stations": "Everest Hub"
+ },
+ {
+ "name": "Col 285 Sector UK-E c12-15",
+ "pos_x": -31.21875,
+ "pos_y": 6.8125,
+ "pos_z": 165.6875,
+ "stations": "Andreas' Folly"
+ },
+ {
+ "name": "Col 285 Sector UK-E c12-28",
+ "pos_x": -51.75,
+ "pos_y": 5.5625,
+ "pos_z": 139.4375,
+ "stations": "Russo Prospect,Plucker's Inheritance"
+ },
+ {
+ "name": "Col 285 Sector UK-E c12-29",
+ "pos_x": -52.96875,
+ "pos_y": -24.6875,
+ "pos_z": 172.875,
+ "stations": "Drake Terminal"
+ },
+ {
+ "name": "Col 285 Sector UO-P c6-15",
+ "pos_x": 111.1875,
+ "pos_y": 114.15625,
+ "pos_z": -80.28125,
+ "stations": "Pullman Palace"
+ },
+ {
+ "name": "Col 285 Sector UO-R d4-83",
+ "pos_x": -59.59375,
+ "pos_y": -177.75,
+ "pos_z": -23.53125,
+ "stations": "Cook Terminal"
+ },
+ {
+ "name": "Col 285 Sector UP-E c12-23",
+ "pos_x": 46.75,
+ "pos_y": 50.8125,
+ "pos_z": 168.9375,
+ "stations": "Baker Town"
+ },
+ {
+ "name": "Col 285 Sector UZ-N c7-8",
+ "pos_x": 30.90625,
+ "pos_y": 162.5,
+ "pos_z": -48.625,
+ "stations": "Rasmussen Installation,Novitski Point"
+ },
+ {
+ "name": "Col 285 Sector UZ-O c6-10",
+ "pos_x": -142.53125,
+ "pos_y": 4.84375,
+ "pos_z": -89.9375,
+ "stations": "Fowler Base,Bass Relay"
+ },
+ {
+ "name": "Col 285 Sector UZ-O c6-12",
+ "pos_x": -144.5,
+ "pos_y": 1.59375,
+ "pos_z": -97.625,
+ "stations": "Rosenberg Horizons"
+ },
+ {
+ "name": "Col 285 Sector UZ-O c6-23",
+ "pos_x": -138.375,
+ "pos_y": 2.78125,
+ "pos_z": -82.40625,
+ "stations": "Marusek Point,Banks Works,Ahern Installation"
+ },
+ {
+ "name": "Col 285 Sector UZ-O c6-9",
+ "pos_x": -144.21875,
+ "pos_y": -12.25,
+ "pos_z": -87.71875,
+ "stations": "Edison Escape"
+ },
+ {
+ "name": "Col 285 Sector UZ-P d5-90",
+ "pos_x": -148.78125,
+ "pos_y": -41.71875,
+ "pos_z": 93.6875,
+ "stations": "Zajdel Landing,Kratman Point"
+ },
+ {
+ "name": "Col 285 Sector VF-E c12-11",
+ "pos_x": -89.15625,
+ "pos_y": -58.46875,
+ "pos_z": 163.34375,
+ "stations": "Hume Base"
+ },
+ {
+ "name": "Col 285 Sector VF-E c12-13",
+ "pos_x": -96.90625,
+ "pos_y": -59.53125,
+ "pos_z": 167.875,
+ "stations": "Moore Base"
+ },
+ {
+ "name": "Col 285 Sector VF-E c12-18",
+ "pos_x": -79.21875,
+ "pos_y": -53,
+ "pos_z": 169.34375,
+ "stations": "Bernoulli Holdings"
+ },
+ {
+ "name": "Col 285 Sector VF-E c12-19",
+ "pos_x": -80.5625,
+ "pos_y": -56.5,
+ "pos_z": 167.75,
+ "stations": "Steakley Arsenal,Nehsi Laboratory"
+ },
+ {
+ "name": "Col 285 Sector VF-E c12-7",
+ "pos_x": -74.5,
+ "pos_y": -63.53125,
+ "pos_z": 160.84375,
+ "stations": "Wiener's Pride"
+ },
+ {
+ "name": "Col 285 Sector VG-I b24-6",
+ "pos_x": -38.4375,
+ "pos_y": -58.0625,
+ "pos_z": 160.8125,
+ "stations": "Wilhelm Point,Nachtigal's Progress"
+ },
+ {
+ "name": "Col 285 Sector VK-N c7-22",
+ "pos_x": -177.71875,
+ "pos_y": 39.65625,
+ "pos_z": -32.28125,
+ "stations": "Scithers' Inheritance"
+ },
+ {
+ "name": "Col 285 Sector VM-N b22-0",
+ "pos_x": -35.03125,
+ "pos_y": 124.21875,
+ "pos_z": 120.78125,
+ "stations": "Sladek Base"
+ },
+ {
+ "name": "Col 285 Sector VO-I c9-12",
+ "pos_x": -13.75,
+ "pos_y": -261.90625,
+ "pos_z": 20.84375,
+ "stations": "Wilhelm von Struve's Folly,Puiseux Prospect"
+ },
+ {
+ "name": "Col 285 Sector VO-I c9-5",
+ "pos_x": -21.21875,
+ "pos_y": -255.5625,
+ "pos_z": 41.875,
+ "stations": "Butler Depot,Hamilton Mines"
+ },
+ {
+ "name": "Col 285 Sector VR-X b16-2",
+ "pos_x": -156.15625,
+ "pos_y": 80.84375,
+ "pos_z": 1.84375,
+ "stations": "Krylov's Progress"
+ },
+ {
+ "name": "Col 285 Sector VS-D a28-4",
+ "pos_x": 152.0625,
+ "pos_y": -25.0625,
+ "pos_z": -50.4375,
+ "stations": "Slade Observatory,Fraas Arsenal"
+ },
+ {
+ "name": "Col 285 Sector VT-W b16-7",
+ "pos_x": 173.28125,
+ "pos_y": -19.1875,
+ "pos_z": 1.3125,
+ "stations": "Egan Installation,Napier Settlement"
+ },
+ {
+ "name": "Col 285 Sector VZ-P d5-69",
+ "pos_x": -129.9375,
+ "pos_y": -38.40625,
+ "pos_z": 91.3125,
+ "stations": "Patterson Refinery"
+ },
+ {
+ "name": "Col 285 Sector WB-R b19-6",
+ "pos_x": -132.3125,
+ "pos_y": -47.15625,
+ "pos_z": 67.8125,
+ "stations": "Goldstein Landing"
+ },
+ {
+ "name": "Col 285 Sector WE-Q d5-106",
+ "pos_x": 162.25,
+ "pos_y": -0.0625,
+ "pos_z": 64.21875,
+ "stations": "RenenBellot Depot,Kuipers Works"
+ },
+ {
+ "name": "Col 285 Sector WE-Q d5-108",
+ "pos_x": 133.21875,
+ "pos_y": 11.34375,
+ "pos_z": 134.71875,
+ "stations": "Marconi Reach"
+ },
+ {
+ "name": "Col 285 Sector WE-Q d5-115",
+ "pos_x": 102.1875,
+ "pos_y": 44.4375,
+ "pos_z": 128.15625,
+ "stations": "Bulgakov Landing,Stiegler Enterprise,Crowley Observatory"
+ },
+ {
+ "name": "Col 285 Sector WE-Q d5-133",
+ "pos_x": 122.0625,
+ "pos_y": 10.34375,
+ "pos_z": 134.9375,
+ "stations": "Romanenko Forum"
+ },
+ {
+ "name": "Col 285 Sector WE-Q d5-137",
+ "pos_x": 137.8125,
+ "pos_y": -22.625,
+ "pos_z": 102.71875,
+ "stations": "Jacobi Enterprise"
+ },
+ {
+ "name": "Col 285 Sector WE-Q d5-140",
+ "pos_x": 156.3125,
+ "pos_y": 32.78125,
+ "pos_z": 104,
+ "stations": "Cole Vision,Andree's Progress"
+ },
+ {
+ "name": "Col 285 Sector WE-Q d5-150",
+ "pos_x": 168.8125,
+ "pos_y": -12.59375,
+ "pos_z": 59.96875,
+ "stations": "Carlisle Hub"
+ },
+ {
+ "name": "Col 285 Sector WE-Q d5-90",
+ "pos_x": 144.84375,
+ "pos_y": 54.71875,
+ "pos_z": 127.8125,
+ "stations": "Wilkes Plant"
+ },
+ {
+ "name": "Col 285 Sector WF-E c12-13",
+ "pos_x": -37.96875,
+ "pos_y": -28.96875,
+ "pos_z": 171.5,
+ "stations": "Malcolm Legacy"
+ },
+ {
+ "name": "Col 285 Sector WF-M c8-11",
+ "pos_x": -38.09375,
+ "pos_y": 172.375,
+ "pos_z": -21.15625,
+ "stations": "Haldeman Beacon"
+ },
+ {
+ "name": "Col 285 Sector WJ-P c6-13",
+ "pos_x": 122.84375,
+ "pos_y": 91.8125,
+ "pos_z": -100.53125,
+ "stations": "Junlong Holdings"
+ },
+ {
+ "name": "Col 285 Sector WO-E b13-0",
+ "pos_x": 136.375,
+ "pos_y": 73.03125,
+ "pos_z": -66.1875,
+ "stations": "Remek Enterprise"
+ },
+ {
+ "name": "Col 285 Sector WO-E b13-1",
+ "pos_x": 140.5,
+ "pos_y": 68.375,
+ "pos_z": -71.5,
+ "stations": "Blackman Enterprise,Macomb Keep,Morris' Claim"
+ },
+ {
+ "name": "Col 285 Sector WP-O d6-101",
+ "pos_x": -38.5625,
+ "pos_y": 58.8125,
+ "pos_z": 161.96875,
+ "stations": "Veblen Market"
+ },
+ {
+ "name": "Col 285 Sector WP-O d6-77",
+ "pos_x": -60.46875,
+ "pos_y": 73.4375,
+ "pos_z": 182.21875,
+ "stations": "Bunch's Folly"
+ },
+ {
+ "name": "Col 285 Sector WQ-J b23-6",
+ "pos_x": -21.09375,
+ "pos_y": -88.96875,
+ "pos_z": 147.34375,
+ "stations": "Pennington Bastion"
+ },
+ {
+ "name": "Col 285 Sector WT-G a40-1",
+ "pos_x": -104.375,
+ "pos_y": -31.78125,
+ "pos_z": 80.5625,
+ "stations": "Fernandez Silo,Shepard Landing"
+ },
+ {
+ "name": "Col 285 Sector WW-O b21-5",
+ "pos_x": -16.0625,
+ "pos_y": 78.34375,
+ "pos_z": 109.625,
+ "stations": "Gantt Barracks"
+ },
+ {
+ "name": "Col 285 Sector WY-F b12-1",
+ "pos_x": 148.6875,
+ "pos_y": 32.65625,
+ "pos_z": -96.9375,
+ "stations": "Barth Market"
+ },
+ {
+ "name": "Col 285 Sector WY-F b12-3",
+ "pos_x": 147.09375,
+ "pos_y": 17.59375,
+ "pos_z": -89.65625,
+ "stations": "Wundt Laboratory,Houtman Vision"
+ },
+ {
+ "name": "Col 285 Sector XA-E c12-23",
+ "pos_x": -65.15625,
+ "pos_y": -71.21875,
+ "pos_z": 160.3125,
+ "stations": "Bacon Terminal"
+ },
+ {
+ "name": "Col 285 Sector XA-E c12-24",
+ "pos_x": -68.28125,
+ "pos_y": -72.5625,
+ "pos_z": 160.1875,
+ "stations": "Nagata Stop"
+ },
+ {
+ "name": "Col 285 Sector XA-E c12-26",
+ "pos_x": -66.09375,
+ "pos_y": -67.28125,
+ "pos_z": 160.34375,
+ "stations": "He's Inheritance,Phillips Installation"
+ },
+ {
+ "name": "Col 285 Sector XA-E c12-27",
+ "pos_x": -71.90625,
+ "pos_y": -68.28125,
+ "pos_z": 161.1875,
+ "stations": "Gresh Prospect"
+ },
+ {
+ "name": "Col 285 Sector XA-E c12-28",
+ "pos_x": -67.4375,
+ "pos_y": -104.71875,
+ "pos_z": 168.78125,
+ "stations": "Peary Works"
+ },
+ {
+ "name": "Col 285 Sector XA-E c12-9",
+ "pos_x": -101,
+ "pos_y": -94.875,
+ "pos_z": 145.0625,
+ "stations": "Grigson Terminal"
+ },
+ {
+ "name": "Col 285 Sector XE-V b17-5",
+ "pos_x": 157.1875,
+ "pos_y": -2.75,
+ "pos_z": 17.78125,
+ "stations": "Burgess Survey,Bagian Refinery,Auld Beacon"
+ },
+ {
+ "name": "Col 285 Sector XF-E c12-12",
+ "pos_x": -2.9375,
+ "pos_y": -46.25,
+ "pos_z": 142.1875,
+ "stations": "Laird Vista,Samuda Holdings"
+ },
+ {
+ "name": "Col 285 Sector XF-N c7-20",
+ "pos_x": -163.0625,
+ "pos_y": 14.03125,
+ "pos_z": -64.5625,
+ "stations": "Wnuk-Lipinski Vision"
+ },
+ {
+ "name": "Col 285 Sector XG-I b24-0",
+ "pos_x": 4.65625,
+ "pos_y": -61.53125,
+ "pos_z": 161.71875,
+ "stations": "Makarov Holdings,Crichton's Progress"
+ },
+ {
+ "name": "Col 285 Sector XJ-P c6-10",
+ "pos_x": 137.03125,
+ "pos_y": 67.5625,
+ "pos_z": -74.1875,
+ "stations": "Lunan Lab"
+ },
+ {
+ "name": "Col 285 Sector XK-E c12-14",
+ "pos_x": 83.9375,
+ "pos_y": 3.40625,
+ "pos_z": 167.53125,
+ "stations": "Penn Terminal"
+ },
+ {
+ "name": "Col 285 Sector XK-E c12-30",
+ "pos_x": 82.375,
+ "pos_y": -20.5625,
+ "pos_z": 163.46875,
+ "stations": "Kazantsev Laboratory"
+ },
+ {
+ "name": "Col 285 Sector XK-E c12-33",
+ "pos_x": 94.625,
+ "pos_y": 1.5625,
+ "pos_z": 148.0625,
+ "stations": "Yu Enterprise"
+ },
+ {
+ "name": "Col 285 Sector XK-O d6-115",
+ "pos_x": -85.5625,
+ "pos_y": 16.25,
+ "pos_z": 162.375,
+ "stations": "Plexico Holdings"
+ },
+ {
+ "name": "Col 285 Sector XK-O d6-48",
+ "pos_x": -121.1875,
+ "pos_y": 7.71875,
+ "pos_z": 152.5625,
+ "stations": "Fisk Vision"
+ },
+ {
+ "name": "Col 285 Sector XK-O d6-56",
+ "pos_x": -75.875,
+ "pos_y": -21.90625,
+ "pos_z": 145.09375,
+ "stations": "Precourt Legacy"
+ },
+ {
+ "name": "Col 285 Sector XK-O d6-70",
+ "pos_x": -81.59375,
+ "pos_y": 43.9375,
+ "pos_z": 157.15625,
+ "stations": "Schroeder Settlement"
+ },
+ {
+ "name": "Col 285 Sector XK-O d6-94",
+ "pos_x": -120,
+ "pos_y": 13.625,
+ "pos_z": 141.78125,
+ "stations": "Ing Landing"
+ },
+ {
+ "name": "Col 285 Sector XK-O d6-95",
+ "pos_x": -110.71875,
+ "pos_y": 21.46875,
+ "pos_z": 151.65625,
+ "stations": "Hawke's Progress,McMullen Point"
+ },
+ {
+ "name": "Col 285 Sector XK-O d6-99",
+ "pos_x": -100.5,
+ "pos_y": 33.03125,
+ "pos_z": 149.6875,
+ "stations": "Griffith Holdings"
+ },
+ {
+ "name": "Col 285 Sector XK-V b16-0",
+ "pos_x": -80.84375,
+ "pos_y": -163.53125,
+ "pos_z": -0.40625,
+ "stations": "Popper Barracks"
+ },
+ {
+ "name": "Col 285 Sector XL-Y b16-1",
+ "pos_x": 47.15625,
+ "pos_y": 155.40625,
+ "pos_z": 4.0625,
+ "stations": "Laumer Legacy"
+ },
+ {
+ "name": "Col 285 Sector XP-O d6-107",
+ "pos_x": 26.9375,
+ "pos_y": 109.1875,
+ "pos_z": 186.5,
+ "stations": "Lasswitz Gateway"
+ },
+ {
+ "name": "Col 285 Sector XP-O d6-109",
+ "pos_x": 20.34375,
+ "pos_y": 113.09375,
+ "pos_z": 185.3125,
+ "stations": "Gessi Installation"
+ },
+ {
+ "name": "Col 285 Sector XP-O d6-56",
+ "pos_x": 39.34375,
+ "pos_y": 83.28125,
+ "pos_z": 174.40625,
+ "stations": "Smith Settlement,Porges Base"
+ },
+ {
+ "name": "Col 285 Sector XP-O d6-58",
+ "pos_x": 25.5,
+ "pos_y": 73.5,
+ "pos_z": 161.65625,
+ "stations": "Birmingham Depot"
+ },
+ {
+ "name": "Col 285 Sector XP-O d6-62",
+ "pos_x": 64.59375,
+ "pos_y": 85.125,
+ "pos_z": 167.21875,
+ "stations": "White's Inheritance"
+ },
+ {
+ "name": "Col 285 Sector XP-O d6-67",
+ "pos_x": 32.3125,
+ "pos_y": 98.125,
+ "pos_z": 191.4375,
+ "stations": "Gaspar de Portola Keep"
+ },
+ {
+ "name": "Col 285 Sector XP-O d6-76",
+ "pos_x": 60.25,
+ "pos_y": 90.09375,
+ "pos_z": 173.03125,
+ "stations": "Hugh Plant"
+ },
+ {
+ "name": "Col 285 Sector XP-O d6-85",
+ "pos_x": 75.1875,
+ "pos_y": 90.25,
+ "pos_z": 152.6875,
+ "stations": "Bolger Works"
+ },
+ {
+ "name": "Col 285 Sector XP-O d6-95",
+ "pos_x": 53.875,
+ "pos_y": 57.65625,
+ "pos_z": 181.5625,
+ "stations": "Leckie's Progress,Gaspar de Portola Reach"
+ },
+ {
+ "name": "Col 285 Sector XP-O d6-96",
+ "pos_x": 57.46875,
+ "pos_y": 58.78125,
+ "pos_z": 187.3125,
+ "stations": "Harrison Holdings,Alexander Terminal,Eskridge Terminal"
+ },
+ {
+ "name": "Col 285 Sector XP-O d6-98",
+ "pos_x": 62.34375,
+ "pos_y": 69.625,
+ "pos_z": 187.21875,
+ "stations": "Alexander Barracks,Beaumont Beacon,Henderson Holdings"
+ },
+ {
+ "name": "Col 285 Sector XT-F b12-2",
+ "pos_x": 131.5,
+ "pos_y": -4.1875,
+ "pos_z": -89.65625,
+ "stations": "Burckhardt Base,Meaney's Inheritance,Borel Settlement"
+ },
+ {
+ "name": "Col 285 Sector XT-G a40-0",
+ "pos_x": -92.3125,
+ "pos_y": -33.90625,
+ "pos_z": 76.8125,
+ "stations": "Staden Vision,Bulleid Vision"
+ },
+ {
+ "name": "Col 285 Sector XU-N c7-12",
+ "pos_x": 77.34375,
+ "pos_y": 105.53125,
+ "pos_z": -63.90625,
+ "stations": "Cantor Vision,Miletus Installation"
+ },
+ {
+ "name": "Col 285 Sector XU-P d5-105",
+ "pos_x": -121.3125,
+ "pos_y": -110.6875,
+ "pos_z": 89.25,
+ "stations": "Cauchy Bastion"
+ },
+ {
+ "name": "Col 285 Sector XU-P d5-79",
+ "pos_x": -114.1875,
+ "pos_y": -106.53125,
+ "pos_z": 129.46875,
+ "stations": "Hamilton Relay"
+ },
+ {
+ "name": "Col 285 Sector XU-P d5-80",
+ "pos_x": -70.5,
+ "pos_y": -182.4375,
+ "pos_z": 92.71875,
+ "stations": "Bendell Stop,Gabriel Settlement"
+ },
+ {
+ "name": "Col 285 Sector XV-L c8-14",
+ "pos_x": -172.84375,
+ "pos_y": 75.375,
+ "pos_z": -21.09375,
+ "stations": "Krusvar Works"
+ },
+ {
+ "name": "Col 285 Sector XV-L c8-18",
+ "pos_x": -161.59375,
+ "pos_y": 79.5,
+ "pos_z": -1.28125,
+ "stations": "Shalatula Base"
+ },
+ {
+ "name": "Col 285 Sector YA-E c12-20",
+ "pos_x": -38.75,
+ "pos_y": -75.21875,
+ "pos_z": 172.90625,
+ "stations": "Eisenstein Laboratory"
+ },
+ {
+ "name": "Col 285 Sector YF-K b10-2",
+ "pos_x": -5.25,
+ "pos_y": 100.28125,
+ "pos_z": -133.1875,
+ "stations": "Cooper Installation,Hackworth Vista"
+ },
+ {
+ "name": "Col 285 Sector YF-M c8-8",
+ "pos_x": 26.8125,
+ "pos_y": 162.78125,
+ "pos_z": -20.1875,
+ "stations": "Lebedev Installation"
+ },
+ {
+ "name": "Col 285 Sector YG-I b24-6",
+ "pos_x": 18.34375,
+ "pos_y": -50.34375,
+ "pos_z": 171.96875,
+ "stations": "Brunel Depot"
+ },
+ {
+ "name": "Col 285 Sector YG-Y b16-1",
+ "pos_x": 16.78125,
+ "pos_y": 138.84375,
+ "pos_z": -2.5,
+ "stations": "The Citadel of Justice"
+ },
+ {
+ "name": "Col 285 Sector YH-N b22-3",
+ "pos_x": -14.46875,
+ "pos_y": 95.03125,
+ "pos_z": 130,
+ "stations": "Cole Refinery"
+ },
+ {
+ "name": "Col 285 Sector YJ-E b13-2",
+ "pos_x": 150.8125,
+ "pos_y": 52.8125,
+ "pos_z": -80.78125,
+ "stations": "Shepard Hub"
+ },
+ {
+ "name": "Col 285 Sector YJ-E b13-3",
+ "pos_x": 150.90625,
+ "pos_y": 47.71875,
+ "pos_z": -72.59375,
+ "stations": "Jones Landing"
+ },
+ {
+ "name": "Col 285 Sector YJ-E b13-4",
+ "pos_x": 151.09375,
+ "pos_y": 41.4375,
+ "pos_z": -76.34375,
+ "stations": "Herbert Depot"
+ },
+ {
+ "name": "Col 285 Sector YK-O d6-109",
+ "pos_x": -5.28125,
+ "pos_y": -8.09375,
+ "pos_z": 173.34375,
+ "stations": "Alvares Landing"
+ },
+ {
+ "name": "Col 285 Sector YK-O d6-135",
+ "pos_x": -33.5625,
+ "pos_y": 32.25,
+ "pos_z": 149.375,
+ "stations": "Hermite Settlement,Collins Refinery,Thompson Horizons"
+ },
+ {
+ "name": "Col 285 Sector YK-O d6-146",
+ "pos_x": -56.78125,
+ "pos_y": 47.09375,
+ "pos_z": 173.59375,
+ "stations": "Hunt Port"
+ },
+ {
+ "name": "Col 285 Sector YK-O d6-147",
+ "pos_x": -20.28125,
+ "pos_y": 10.90625,
+ "pos_z": 158.21875,
+ "stations": "Barbaro Vision"
+ },
+ {
+ "name": "Col 285 Sector YK-O d6-151",
+ "pos_x": -64.8125,
+ "pos_y": -0.1875,
+ "pos_z": 141.3125,
+ "stations": "Meyrink Silo,Merle Base"
+ },
+ {
+ "name": "Col 285 Sector YK-O d6-73",
+ "pos_x": -51.28125,
+ "pos_y": 32.53125,
+ "pos_z": 147.21875,
+ "stations": "Fernao do Po Silo,Hardy Relay,Bethke Horizons"
+ },
+ {
+ "name": "Col 285 Sector YT-F b12-3",
+ "pos_x": 135.34375,
+ "pos_y": -0.9375,
+ "pos_z": -90.96875,
+ "stations": "Slonczewski Escape,Raleigh Bastion"
+ },
+ {
+ "name": "Col 285 Sector YU-F c11-1",
+ "pos_x": 98.53125,
+ "pos_y": -92.625,
+ "pos_z": 97.625,
+ "stations": "The Sepulchre"
+ },
+ {
+ "name": "Col 285 Sector YU-N c7-8",
+ "pos_x": 109.71875,
+ "pos_y": 118.78125,
+ "pos_z": -62.9375,
+ "stations": "Ricci Laboratory"
+ },
+ {
+ "name": "Col 285 Sector YV-L c8-6",
+ "pos_x": -141.09375,
+ "pos_y": 85.125,
+ "pos_z": -17.96875,
+ "stations": "Alexander Vision"
+ },
+ {
+ "name": "Col 285 Sector YZ-C b14-1",
+ "pos_x": 147.4375,
+ "pos_y": 76.21875,
+ "pos_z": -58.46875,
+ "stations": "Stross Keep"
+ },
+ {
+ "name": "Col 285 Sector YZ-P d5-127",
+ "pos_x": 137.28125,
+ "pos_y": -34.9375,
+ "pos_z": 109.6875,
+ "stations": "Cassini Keep"
+ },
+ {
+ "name": "Col 285 Sector ZB-R b19-2",
+ "pos_x": -81.0625,
+ "pos_y": -63.28125,
+ "pos_z": 61.53125,
+ "stations": "Ore Observatory,Bradshaw Survey,Keyes Landing,Sheffield's Folly"
+ },
+ {
+ "name": "Col 285 Sector ZE-P c6-11",
+ "pos_x": 137.5,
+ "pos_y": 34.21875,
+ "pos_z": -95.3125,
+ "stations": "Wilder Enterprise"
+ },
+ {
+ "name": "Col 285 Sector ZE-P c6-21",
+ "pos_x": 159.5,
+ "pos_y": 36.28125,
+ "pos_z": -92.0625,
+ "stations": "Lichtenberg Works"
+ },
+ {
+ "name": "Col 285 Sector ZF-E c12-13",
+ "pos_x": 91.125,
+ "pos_y": -45.125,
+ "pos_z": 159.1875,
+ "stations": "Besonders Prospect"
+ },
+ {
+ "name": "Col 285 Sector ZF-E c12-15",
+ "pos_x": 84.90625,
+ "pos_y": -45.0625,
+ "pos_z": 162.78125,
+ "stations": "Beliaev Lab"
+ },
+ {
+ "name": "Col 285 Sector ZF-E c12-20",
+ "pos_x": 91.40625,
+ "pos_y": -32.5625,
+ "pos_z": 173.0625,
+ "stations": "Rowley Depot"
+ },
+ {
+ "name": "Col 285 Sector ZF-O d6-114",
+ "pos_x": -84.84375,
+ "pos_y": -49,
+ "pos_z": 147.46875,
+ "stations": "Dashiell Barracks"
+ },
+ {
+ "name": "Col 285 Sector ZF-O d6-115",
+ "pos_x": -90.375,
+ "pos_y": -36.34375,
+ "pos_z": 155.375,
+ "stations": "Rand Holdings"
+ },
+ {
+ "name": "Col 285 Sector ZF-O d6-60",
+ "pos_x": -87.3125,
+ "pos_y": -57.78125,
+ "pos_z": 175.375,
+ "stations": "Pavlou Depot"
+ },
+ {
+ "name": "Col 285 Sector ZF-O d6-62",
+ "pos_x": -86.65625,
+ "pos_y": -54.3125,
+ "pos_z": 147.15625,
+ "stations": "Grzimek Refinery"
+ },
+ {
+ "name": "Col 285 Sector ZF-O d6-67",
+ "pos_x": -91.96875,
+ "pos_y": -25.1875,
+ "pos_z": 146.03125,
+ "stations": "Tilman Beacon"
+ },
+ {
+ "name": "Col 285 Sector ZK-O d6-137",
+ "pos_x": 61.5625,
+ "pos_y": 37.09375,
+ "pos_z": 194.78125,
+ "stations": "Everest's Inheritance"
+ },
+ {
+ "name": "Col 285 Sector ZK-O d6-87",
+ "pos_x": 89.0625,
+ "pos_y": 37.34375,
+ "pos_z": 154.375,
+ "stations": "Rzeppa Beacon,Reynolds Penal colony"
+ },
+ {
+ "name": "Col 285 Sector ZL-K c9-9",
+ "pos_x": -85.6875,
+ "pos_y": 145.84375,
+ "pos_z": 38.65625,
+ "stations": "Bursch Installation"
+ },
+ {
+ "name": "Col 285 Sector ZQ-L c8-26",
+ "pos_x": -160.625,
+ "pos_y": 19.875,
+ "pos_z": -13.8125,
+ "stations": "Tall Point"
+ },
+ {
+ "name": "Col 285 Sector ZQ-L c8-8",
+ "pos_x": -158.09375,
+ "pos_y": 20.125,
+ "pos_z": -8.34375,
+ "stations": "Akers Arsenal,Turzillo Horizons"
+ },
+ {
+ "name": "Col 285 Sector ZS-M b22-1",
+ "pos_x": -116.03125,
+ "pos_y": 52.46875,
+ "pos_z": 124.53125,
+ "stations": "H. G. Wells Horizons"
+ },
+ {
+ "name": "Col 285 Sector ZU-C b14-1",
+ "pos_x": 116,
+ "pos_y": 61.9375,
+ "pos_z": -62.25,
+ "stations": "Grothendieck Camp"
+ },
+ {
+ "name": "Col 285 Sector ZX-V b17-3",
+ "pos_x": -148.96875,
+ "pos_y": 75.0625,
+ "pos_z": 20.65625,
+ "stations": "Ellison Depot"
+ },
+ {
+ "name": "Colabri",
+ "pos_x": 150.09375,
+ "pos_y": 81,
+ "pos_z": 12.21875,
+ "stations": "Bellamy Dock,Watts Freeport"
+ },
+ {
+ "name": "Colai",
+ "pos_x": -148.5625,
+ "pos_y": 6.34375,
+ "pos_z": 79.25,
+ "stations": "Cockrell Dock,Kizim Port,Curbeam Settlement,Fourier Depot,Mallory Works"
+ },
+ {
+ "name": "Colando Po",
+ "pos_x": 94.28125,
+ "pos_y": 43.5625,
+ "pos_z": 70.8125,
+ "stations": "Clifford Terminal"
+ },
+ {
+ "name": "Colanja",
+ "pos_x": -18.15625,
+ "pos_y": 8.875,
+ "pos_z": 12.25,
+ "stations": "Garriott Settlement,Teng-hui Landing,Watson Horizons,Vishweswarayya Vision"
+ },
+ {
+ "name": "Collangbaka",
+ "pos_x": 38.46875,
+ "pos_y": -62.28125,
+ "pos_z": -32,
+ "stations": "Pennington Hangar,Vaugh Port,Drake Bastion"
+ },
+ {
+ "name": "Collottung",
+ "pos_x": 68.875,
+ "pos_y": -92.25,
+ "pos_z": -73.5625,
+ "stations": "Hickman Hub"
+ },
+ {
+ "name": "Colonia",
+ "pos_x": -9530.5,
+ "pos_y": -910.28125,
+ "pos_z": 19808.125,
+ "stations": "Jaques Station,Colonia Hub,Colonia Orbital,Dove Enigma"
+ },
+ {
+ "name": "Coma",
+ "pos_x": 86,
+ "pos_y": -15.46875,
+ "pos_z": 23.28125,
+ "stations": "Ackerman Depot,Malenchenko City,So-yeon Hub,Alcala Port"
+ },
+ {
+ "name": "Comam",
+ "pos_x": 31.96875,
+ "pos_y": -24.125,
+ "pos_z": 147.15625,
+ "stations": "Bolotov Vision,Nikolayev Prospect,Dobzhansky Stop"
+ },
+ {
+ "name": "Coman",
+ "pos_x": 78.375,
+ "pos_y": -157.03125,
+ "pos_z": -21.1875,
+ "stations": "Lomonosov Retreat,Sunyaev Survey"
+ },
+ {
+ "name": "Comana",
+ "pos_x": -108.59375,
+ "pos_y": 21.15625,
+ "pos_z": 98.0625,
+ "stations": "Gaspar de Portola Dock,Wright Penal colony,Rond d'Alembert Vision,Kneale Dock"
+ },
+ {
+ "name": "Comane",
+ "pos_x": 27.09375,
+ "pos_y": -90.90625,
+ "pos_z": -112.65625,
+ "stations": "Piaget Base,May Estate,Huberath Hub"
+ },
+ {
+ "name": "Comanes",
+ "pos_x": 90.3125,
+ "pos_y": -97.125,
+ "pos_z": -33.1875,
+ "stations": "Hirn Terminal,Huberath Works,Al Sufi Terminal,Langsdorff Holdings"
+ },
+ {
+ "name": "Comanga",
+ "pos_x": -91.1875,
+ "pos_y": -69.65625,
+ "pos_z": 60.875,
+ "stations": "Henry Port,Ramsbottom Port,Butz Station,Reilly Dock,Garan Keep,Haller Hub,Hopper Station,Champlain Town,Kuttner Terminal,Boulton Horizons,Bohm's Inheritance,Gibson Penal colony,Cabot Base"
+ },
+ {
+ "name": "Comasci",
+ "pos_x": 170.46875,
+ "pos_y": -118.21875,
+ "pos_z": 77.875,
+ "stations": "Oshima Platform,Filter Installation"
+ },
+ {
+ "name": "Comaucate",
+ "pos_x": 65.28125,
+ "pos_y": -197.625,
+ "pos_z": 62,
+ "stations": "Kahn's Folly,Wenzel Platform,Merritt Base"
+ },
+ {
+ "name": "Comecab",
+ "pos_x": 97.6875,
+ "pos_y": -43.375,
+ "pos_z": 67.84375,
+ "stations": "Thierree Enterprise"
+ },
+ {
+ "name": "Concani",
+ "pos_x": -60.53125,
+ "pos_y": 135.40625,
+ "pos_z": 16.53125,
+ "stations": "Grant Enterprise,Hayden Co-operative,Bierce Station,Watts Vision"
+ },
+ {
+ "name": "Concantae",
+ "pos_x": 103.125,
+ "pos_y": -85.59375,
+ "pos_z": -68.09375,
+ "stations": "Tietjen Ring,Hodgkinson Beacon,Narlikar Hub,Shoujing Hub"
+ },
+ {
+ "name": "Concateneg",
+ "pos_x": 9.375,
+ "pos_y": -112.375,
+ "pos_z": 112.5,
+ "stations": "Kraft Terminal"
+ },
+ {
+ "name": "Concheliga",
+ "pos_x": 23,
+ "pos_y": 26.21875,
+ "pos_z": -175.75,
+ "stations": "Littlewood Orbital,Henderson Base,Bertin's Inheritance"
+ },
+ {
+ "name": "Conchucos",
+ "pos_x": 29.15625,
+ "pos_y": -58.125,
+ "pos_z": 81.21875,
+ "stations": "Bereznyak Dock,Goulart Landing,Arisman Hub,Brule Bastion"
+ },
+ {
+ "name": "Condani",
+ "pos_x": -109.5625,
+ "pos_y": 131.53125,
+ "pos_z": -36.46875,
+ "stations": "Matheson Terminal,Rey Settlement,Boucher Hub"
+ },
+ {
+ "name": "Condharwal",
+ "pos_x": -15.09375,
+ "pos_y": 14.9375,
+ "pos_z": -81.25,
+ "stations": "Hodgkin Port,Piercy Horizons,Allen's Inheritance"
+ },
+ {
+ "name": "Condi",
+ "pos_x": 106.71875,
+ "pos_y": -16.78125,
+ "pos_z": 37.21875,
+ "stations": "Crouch Depot,Gemar Station,Sherrington Terminal,Ray Station,Reynolds Installation"
+ },
+ {
+ "name": "Condovichs",
+ "pos_x": 64.9375,
+ "pos_y": -93.53125,
+ "pos_z": 25.46875,
+ "stations": "Walters Port"
+ },
+ {
+ "name": "Coni",
+ "pos_x": 91.90625,
+ "pos_y": -59.75,
+ "pos_z": -36.375,
+ "stations": "Jewitt Landing,Parker Gateway,Nahavandi's Claim,Marcy Terminal"
+ },
+ {
+ "name": "Coniaci",
+ "pos_x": 174.71875,
+ "pos_y": -155.625,
+ "pos_z": 114.34375,
+ "stations": "Froud Outpost"
+ },
+ {
+ "name": "Conian",
+ "pos_x": -105.6875,
+ "pos_y": -17.59375,
+ "pos_z": 117.65625,
+ "stations": "Andersson's Progress"
+ },
+ {
+ "name": "Conii",
+ "pos_x": 96.65625,
+ "pos_y": -172.75,
+ "pos_z": 135.25,
+ "stations": "Lavochkin Platform,Morgan Point"
+ },
+ {
+ "name": "Conneda",
+ "pos_x": -10.5625,
+ "pos_y": -3.65625,
+ "pos_z": 41.75,
+ "stations": "Fiennes Barracks,Brahe Station,Illy Refinery"
+ },
+ {
+ "name": "Contegus",
+ "pos_x": -81.8125,
+ "pos_y": -15.8125,
+ "pos_z": 39.1875,
+ "stations": "Wang Dock"
+ },
+ {
+ "name": "Contie",
+ "pos_x": -3.1875,
+ "pos_y": -196.75,
+ "pos_z": 43.03125,
+ "stations": "Shajn Platform,Patterson Horizons"
+ },
+ {
+ "name": "Contien",
+ "pos_x": 41.125,
+ "pos_y": 13.5625,
+ "pos_z": 109.9375,
+ "stations": "Eanes Vision,Legendre Survey,Stevens Vision,Einstein Lab"
+ },
+ {
+ "name": "Contii",
+ "pos_x": -80.5625,
+ "pos_y": -23.75,
+ "pos_z": 119.59375,
+ "stations": "Griffith Gateway,Kent Barracks,Celebi City,Wiener Vision,Sucharitkul Vision,Levi-Civita Orbital,Garnier Enterprise,Capek Base"
+ },
+ {
+ "name": "Contiku",
+ "pos_x": -16.0625,
+ "pos_y": -143.09375,
+ "pos_z": 59.03125,
+ "stations": "Jansky Vision,Swift Station,Maire Settlement,Ritchey Terminal"
+ },
+ {
+ "name": "Conven",
+ "pos_x": -7.5625,
+ "pos_y": -96.71875,
+ "pos_z": 164.46875,
+ "stations": "Miletus Platform,Serre Legacy,Phillpotts' Progress"
+ },
+ {
+ "name": "Conventes",
+ "pos_x": 1.03125,
+ "pos_y": 106.78125,
+ "pos_z": -64.03125,
+ "stations": "Delany Station,Ordway Plant"
+ },
+ {
+ "name": "Convoumia",
+ "pos_x": 41.125,
+ "pos_y": -5.9375,
+ "pos_z": 13.15625,
+ "stations": "Arnold Hub,Willis Prospect,Savery Base,Hornby Horizons"
+ },
+ {
+ "name": "Convoumis",
+ "pos_x": 151.40625,
+ "pos_y": -65.90625,
+ "pos_z": 53.65625,
+ "stations": "Pittendreigh Oasis,Regiomontanus Holdings"
+ },
+ {
+ "name": "Cook",
+ "pos_x": 142.28125,
+ "pos_y": -66.40625,
+ "pos_z": 27.875,
+ "stations": "Bohr Terminal,Eisinga Colony,Wilson Port,Medupe Hub"
+ },
+ {
+ "name": "Cook Odua",
+ "pos_x": 47.71875,
+ "pos_y": -33.96875,
+ "pos_z": 142.75,
+ "stations": "Jones Base,Ivins Works,Jemison Works"
+ },
+ {
+ "name": "Copian Xing",
+ "pos_x": -83.65625,
+ "pos_y": -26.8125,
+ "pos_z": -45.21875,
+ "stations": "Akiyama Enterprise"
+ },
+ {
+ "name": "Copitanii",
+ "pos_x": 104.8125,
+ "pos_y": -42.84375,
+ "pos_z": 31.125,
+ "stations": "Bauman Vision"
+ },
+ {
+ "name": "Copityar",
+ "pos_x": 122.53125,
+ "pos_y": -61.09375,
+ "pos_z": -49.125,
+ "stations": "Herzog Orbital,Darwin Landing"
+ },
+ {
+ "name": "Coqualki",
+ "pos_x": 144.5625,
+ "pos_y": -3.75,
+ "pos_z": 72.90625,
+ "stations": "Caselberg Freeport,Strzelecki Bastion,Obruchev's Claim"
+ },
+ {
+ "name": "Coqueelgovi",
+ "pos_x": -10.8125,
+ "pos_y": -138.59375,
+ "pos_z": 97.125,
+ "stations": "Shunkai Terminal,Pickering Installation"
+ },
+ {
+ "name": "Coquenchel",
+ "pos_x": -43.6875,
+ "pos_y": 110.46875,
+ "pos_z": 38.3125,
+ "stations": "Rice Vision,Jeschke Estate,Dyomin Bastion,Womack Holdings"
+ },
+ {
+ "name": "Coquessa",
+ "pos_x": -66.65625,
+ "pos_y": -55.78125,
+ "pos_z": -12.4375,
+ "stations": "Fung Point,Musgrave Works"
+ },
+ {
+ "name": "Coqui Renes",
+ "pos_x": -102.1875,
+ "pos_y": 20.1875,
+ "pos_z": -23.125,
+ "stations": "Hooke Ring,Kirchoff Enterprise,Fullerton Hub,McKee Point"
+ },
+ {
+ "name": "Coqui Xee",
+ "pos_x": 23.84375,
+ "pos_y": -70.3125,
+ "pos_z": -44.46875,
+ "stations": "Bell City,Gardner Base,Durrance Hangar,Adams' Folly,Mullane Platform"
+ },
+ {
+ "name": "Coquiabayo",
+ "pos_x": 121.90625,
+ "pos_y": -45.0625,
+ "pos_z": 80,
+ "stations": "Kubokawa Dock,Kelly Terminal,Whittle Vision"
+ },
+ {
+ "name": "Coquim",
+ "pos_x": 20.75,
+ "pos_y": -82.25,
+ "pos_z": 33.59375,
+ "stations": "Hirayama Installation,Phillips Hub,Evans Settlement,Sarrantonio Bastion"
+ },
+ {
+ "name": "Cor Caroli",
+ "pos_x": -19.78125,
+ "pos_y": 112.6875,
+ "pos_z": -9.5625,
+ "stations": "Stephenson Station"
+ },
+ {
+ "name": "Coras",
+ "pos_x": 40.34375,
+ "pos_y": -131.84375,
+ "pos_z": -33.875,
+ "stations": "Berkey Works,Lindblad Landing,Narlikar Dock"
+ },
+ {
+ "name": "Coratha",
+ "pos_x": 72.25,
+ "pos_y": 111.59375,
+ "pos_z": 56.78125,
+ "stations": "Korzun Ring,Gohar Gateway,Braun City,Jiushao Vision,Soddy Bastion"
+ },
+ {
+ "name": "Corbago",
+ "pos_x": -88,
+ "pos_y": -65.40625,
+ "pos_z": 115.75,
+ "stations": "Kafka Terminal,Pratchett Hub,Fadlan Dock"
+ },
+ {
+ "name": "Corbenic",
+ "pos_x": 35.71875,
+ "pos_y": 1.5,
+ "pos_z": -6.4375,
+ "stations": "Hertz City,White Depot,Fullerton Terminal,Fermi Landing"
+ },
+ {
+ "name": "Corbin",
+ "pos_x": -32.09375,
+ "pos_y": -10.25,
+ "pos_z": -15.09375,
+ "stations": "Illy City,Dyson Dock,Wundt Terminal,Midgley Hub,Kovalevsky Terminal,Savinykh City,Hopkins Dock,Mukai Depot"
+ },
+ {
+ "name": "Cordenet",
+ "pos_x": 60.9375,
+ "pos_y": -70.5625,
+ "pos_z": 28.5625,
+ "stations": "Chernykh Station,Tago Colony,Brom Hub,Karachkina Vision,Moorcock's Progress,Leberecht Tempel Gateway"
+ },
+ {
+ "name": "Cordenses",
+ "pos_x": 158.65625,
+ "pos_y": -10.15625,
+ "pos_z": -50.6875,
+ "stations": "Wylie Co-operative,Harris Terminal,Ballard Works,Kirk Enterprise,Brooks Base"
+ },
+ {
+ "name": "Coriabog",
+ "pos_x": -55.53125,
+ "pos_y": -61.34375,
+ "pos_z": 69.0625,
+ "stations": "Carter Enterprise,Tryggvason Terminal,Heisenberg Terminal,Rice Silo"
+ },
+ {
+ "name": "Corian Guit",
+ "pos_x": -162.9375,
+ "pos_y": 25.125,
+ "pos_z": 72.53125,
+ "stations": "Belyanin City,Springer Port,Cousteau Colony,Fourier Landing,Walters Base"
+ },
+ {
+ "name": "Coriat",
+ "pos_x": 46.6875,
+ "pos_y": -226,
+ "pos_z": 71,
+ "stations": "Cellarius Dock,Pu's Progress,Jael Vision,Kerimov Station"
+ },
+ {
+ "name": "Coriba",
+ "pos_x": 156.40625,
+ "pos_y": -160.5625,
+ "pos_z": -22.6875,
+ "stations": "Ikeya Beacon"
+ },
+ {
+ "name": "Coribes",
+ "pos_x": 158.09375,
+ "pos_y": 8.125,
+ "pos_z": -3.21875,
+ "stations": "Georg Bothe Gateway,Kaleri Hub,Lonchakov Horizons"
+ },
+ {
+ "name": "Coriccha",
+ "pos_x": -14.40625,
+ "pos_y": 19.9375,
+ "pos_z": 7,
+ "stations": "Schirra Dock,Beckman Station,Mendeleev Gateway,Rushd City,Tanner City,Gurney Orbital"
+ },
+ {
+ "name": "Corin",
+ "pos_x": 95.71875,
+ "pos_y": -116.4375,
+ "pos_z": 100.75,
+ "stations": "Nomen Port,Fowler Market,Seki Vision,Andrews Prospect,Lassell Station,Sandage City,Paola Dock,Edwards Forum,Ing Point,Wallerstein Hub"
+ },
+ {
+ "name": "Coringha",
+ "pos_x": 118.40625,
+ "pos_y": -84.75,
+ "pos_z": 9.0625,
+ "stations": "Riebe Camp,Green Keep"
+ },
+ {
+ "name": "Corio",
+ "pos_x": 138.59375,
+ "pos_y": -141.96875,
+ "pos_z": -39.875,
+ "stations": "Kagawa Holdings,Darwin City,Bouvard Port"
+ },
+ {
+ "name": "Corioru",
+ "pos_x": 116.3125,
+ "pos_y": -87.4375,
+ "pos_z": -45.28125,
+ "stations": "Sleator Port,Markham Enterprise,Hamilton Station,Anderson Works"
+ },
+ {
+ "name": "Corioskur",
+ "pos_x": 107.78125,
+ "pos_y": -91.15625,
+ "pos_z": -46.46875,
+ "stations": "Tombaugh Port,Rigaux Settlement,Ackerman Settlement"
+ },
+ {
+ "name": "Coriosolite",
+ "pos_x": -134.09375,
+ "pos_y": -34.75,
+ "pos_z": -93.375,
+ "stations": "Stillman Landing"
+ },
+ {
+ "name": "Corisci",
+ "pos_x": 57.5625,
+ "pos_y": 34.28125,
+ "pos_z": 117.6875,
+ "stations": "Liebig Port,Blodgett Relay"
+ },
+ {
+ "name": "Coritab",
+ "pos_x": -33.6875,
+ "pos_y": -41.3125,
+ "pos_z": 102.84375,
+ "stations": "Freud Gateway"
+ },
+ {
+ "name": "Corithi",
+ "pos_x": -157.5,
+ "pos_y": 29.90625,
+ "pos_z": 28.5,
+ "stations": "Auld Platform"
+ },
+ {
+ "name": "Coritini",
+ "pos_x": -117.4375,
+ "pos_y": 117,
+ "pos_z": -64.28125,
+ "stations": "Spinrad Port,Chaviano Station,Moon Hub,Clarke Keep"
+ },
+ {
+ "name": "Coritures",
+ "pos_x": 105.6875,
+ "pos_y": 46.5625,
+ "pos_z": 104.96875,
+ "stations": "Stith Mines"
+ },
+ {
+ "name": "Corn Pin",
+ "pos_x": 7.9375,
+ "pos_y": -4.71875,
+ "pos_z": 83.3125,
+ "stations": "Boodt Terminal,Wellman Orbital"
+ },
+ {
+ "name": "Cornacates",
+ "pos_x": 150.65625,
+ "pos_y": -90.375,
+ "pos_z": 47.59375,
+ "stations": "Hulse Landing,Trumpler Vista,Villarceau Vision"
+ },
+ {
+ "name": "Cornae",
+ "pos_x": 59.125,
+ "pos_y": -174.875,
+ "pos_z": -49.6875,
+ "stations": "Beshore Dock,Shavyrin Survey,Marriott Works"
+ },
+ {
+ "name": "Cornengu",
+ "pos_x": 113.34375,
+ "pos_y": -18.125,
+ "pos_z": -150.9375,
+ "stations": "Margulies Dock,Staden Dock,Hamilton Survey,Pike Enterprise,Kessel Terminal"
+ },
+ {
+ "name": "Corngaranga",
+ "pos_x": -47.21875,
+ "pos_y": 82.03125,
+ "pos_z": -9.28125,
+ "stations": "Teng Hub,Littlewood Dock"
+ },
+ {
+ "name": "Corngari",
+ "pos_x": 66.09375,
+ "pos_y": -27.125,
+ "pos_z": 68.40625,
+ "stations": "Gould Station,Tedin Station,Napier's Inheritance"
+ },
+ {
+ "name": "Corngi",
+ "pos_x": -0.1875,
+ "pos_y": -134.8125,
+ "pos_z": 118.90625,
+ "stations": "Bartini Base"
+ },
+ {
+ "name": "Cornsar",
+ "pos_x": -94.15625,
+ "pos_y": -53.5625,
+ "pos_z": -15.90625,
+ "stations": "Ito Market,Chadwick Port,Poincare Silo,Fleming Arena,Brunel Laboratory"
+ },
+ {
+ "name": "Corycia",
+ "pos_x": 19.375,
+ "pos_y": 6.34375,
+ "pos_z": 43.5,
+ "stations": "Piper Hub,Lasswitz Hub,Armstrong Port,Drexler Hub,Paez Hub"
+ },
+ {
+ "name": "Cosi",
+ "pos_x": 24.75,
+ "pos_y": -14.6875,
+ "pos_z": -70.75,
+ "stations": "Ising Hub,Arrhenius Hub,Julian City,Crumey Legacy,Solovyov Orbital,Zettel Refinery,Worlidge Station,Bogdanov Base,Henry Hub,Marshall Hub"
+ },
+ {
+ "name": "Cosiao",
+ "pos_x": 149.875,
+ "pos_y": -66.6875,
+ "pos_z": -21.75,
+ "stations": "Chacornac Terminal,Kent Arsenal"
+ },
+ {
+ "name": "Cotiachis",
+ "pos_x": -8.15625,
+ "pos_y": -164.78125,
+ "pos_z": 75.40625,
+ "stations": "Leberecht Tempel City,Cayley City"
+ },
+ {
+ "name": "Cotidji",
+ "pos_x": 22.96875,
+ "pos_y": -17.5625,
+ "pos_z": -134.5,
+ "stations": "Dayuan Co-operative,Kovalevskaya Co-operative,Penn Enterprise,Buffett Vision,Roddenberry Survey"
+ },
+ {
+ "name": "Cotigagaial",
+ "pos_x": 62.09375,
+ "pos_y": 121.6875,
+ "pos_z": -24.4375,
+ "stations": "Ali Port,Divis Enterprise,Wescott Hub,Holdstock Prospect,Bose Station,Shosuke Oasis,Thornton Station,Nye Port,Gaffney Dock,Northrop Landing,Wood Installation"
+ },
+ {
+ "name": "Cotiges",
+ "pos_x": -107.8125,
+ "pos_y": -68.09375,
+ "pos_z": 52.9375,
+ "stations": "Haxel Stop"
+ },
+ {
+ "name": "Cotin",
+ "pos_x": 16.875,
+ "pos_y": -17.875,
+ "pos_z": 167.34375,
+ "stations": "Markham Depot,Bishop Mines"
+ },
+ {
+ "name": "Cotine",
+ "pos_x": 154.59375,
+ "pos_y": 40.125,
+ "pos_z": 55.71875,
+ "stations": "Darboux Landing"
+ },
+ {
+ "name": "Cotini",
+ "pos_x": -83,
+ "pos_y": -38.28125,
+ "pos_z": 77.46875,
+ "stations": "Fraley Relay"
+ },
+ {
+ "name": "Cowicas",
+ "pos_x": -90.65625,
+ "pos_y": 109.65625,
+ "pos_z": 15.375,
+ "stations": "Moriarty Dock,Harrison Station,Goonan Enterprise,Clair Enterprise,Milnor Terminal,al-Khowarizmi Dock"
+ },
+ {
+ "name": "Cowini",
+ "pos_x": 64.59375,
+ "pos_y": -146.6875,
+ "pos_z": 100,
+ "stations": "Havilland Dock,Calatrava Station,Shute Dock,Kandrup Hub,Tombaugh Terminal,Russell Dock,Chorel Plant,Secchi Hub,Takahashi Station,Hiyya Port,Mori Survey"
+ },
+ {
+ "name": "Cowiska",
+ "pos_x": -102.90625,
+ "pos_y": -22.21875,
+ "pos_z": 102.84375,
+ "stations": "Gabriel Arsenal,Remec Dock,Larbalestier Beacon"
+ },
+ {
+ "name": "Cowiskimo",
+ "pos_x": 162.71875,
+ "pos_y": 48.8125,
+ "pos_z": 15.53125,
+ "stations": "Markov Co-operative"
+ },
+ {
+ "name": "Coyopa",
+ "pos_x": 114.3125,
+ "pos_y": -99.875,
+ "pos_z": -104.75,
+ "stations": "Minkowski Colony,Yerka Platform,Coddington Colony,Chorel Vision"
+ },
+ {
+ "name": "Coyotani",
+ "pos_x": 174.0625,
+ "pos_y": -71.21875,
+ "pos_z": 124.78125,
+ "stations": "Oberth Dock,William Sargent Port,Bischoff Vision"
+ },
+ {
+ "name": "Cozaanat",
+ "pos_x": 51.1875,
+ "pos_y": -189.40625,
+ "pos_z": -37.03125,
+ "stations": "Stiegler Survey,Ejigu Survey,Druillet Colony,Robert Terminal"
+ },
+ {
+ "name": "CPC 20 6743",
+ "pos_x": 23.71875,
+ "pos_y": -55.90625,
+ "pos_z": 54.9375,
+ "stations": "Searfoss Vision,Lyakhov Dock,Aksyonov Keep,Fullerton Arena"
+ },
+ {
+ "name": "CPC 22 212",
+ "pos_x": 69.8125,
+ "pos_y": -58.625,
+ "pos_z": 40.3125,
+ "stations": "Roed Odegaard City,Rechtin Gateway,Encke Orbital,Theodor Winnecke Lab,Goonan Beacon"
+ },
+ {
+ "name": "CPD-23 501",
+ "pos_x": 60.59375,
+ "pos_y": 35.3125,
+ "pos_z": 0.625,
+ "stations": "Malchiodi City"
+ },
+ {
+ "name": "CPD-28 332",
+ "pos_x": 9.8125,
+ "pos_y": -24.46875,
+ "pos_z": -10.65625,
+ "stations": "Hopkins Station,Lebedev Hub,Winne Gateway,Willis Prospect"
+ },
+ {
+ "name": "CPD-51 3323",
+ "pos_x": 76.125,
+ "pos_y": 6.90625,
+ "pos_z": 16.1875,
+ "stations": "Tuan Dock,Brand Barracks"
+ },
+ {
+ "name": "CPD-59 314",
+ "pos_x": 61.53125,
+ "pos_y": -58.15625,
+ "pos_z": 0.78125,
+ "stations": "Helin Hub,Caselberg Relay,Holdstock Observatory"
+ },
+ {
+ "name": "CPD-60 604",
+ "pos_x": 69.25,
+ "pos_y": -34.9375,
+ "pos_z": -0.65625,
+ "stations": "Melvin Port,Gantt Enterprise,Garrett Landing"
+ },
+ {
+ "name": "CPD-64 4178",
+ "pos_x": 35.6875,
+ "pos_y": -64.21875,
+ "pos_z": 56.59375,
+ "stations": "Rocklynne Hub"
+ },
+ {
+ "name": "CPD-70 2439",
+ "pos_x": 43.8125,
+ "pos_y": -28.90625,
+ "pos_z": 59,
+ "stations": "Weyn Dock,Szentmartony Dock,Fossum Keep,Godwin Dock"
+ },
+ {
+ "name": "CPO 24",
+ "pos_x": 16.28125,
+ "pos_y": -75.9375,
+ "pos_z": 9.21875,
+ "stations": "Grabe Hangar,Heng Terminal"
+ },
+ {
+ "name": "CR Draco",
+ "pos_x": -49.46875,
+ "pos_y": 47.4375,
+ "pos_z": 4.375,
+ "stations": "Semeonis Settlement,Celebi Hub,Glidden Vision"
+ },
+ {
+ "name": "CR Draconis",
+ "pos_x": -48.3125,
+ "pos_y": 46.15625,
+ "pos_z": 4.46875,
+ "stations": "Andreas Horizons"
+ },
+ {
+ "name": "Crab Sector DL-Y d9",
+ "pos_x": 559.625,
+ "pos_y": -708.0625,
+ "pos_z": -6947.5625,
+ "stations": "Station X"
+ },
+ {
+ "name": "Cratanakulu",
+ "pos_x": -93.84375,
+ "pos_y": -102.5625,
+ "pos_z": -42.71875,
+ "stations": "Sekowski Landing"
+ },
+ {
+ "name": "Crataru",
+ "pos_x": -57.1875,
+ "pos_y": 84.125,
+ "pos_z": -122.1875,
+ "stations": "Palmer Survey,Humphreys Landing,Taine Point"
+ },
+ {
+ "name": "Crathajapus",
+ "pos_x": -103.875,
+ "pos_y": -124.53125,
+ "pos_z": 20.71875,
+ "stations": "Daley City,Godwin Station,Bradbury Silo,Bisson Observatory"
+ },
+ {
+ "name": "Crathiganit",
+ "pos_x": 29.71875,
+ "pos_y": -18.59375,
+ "pos_z": -101.3125,
+ "stations": "Resnick Market,Iqbal Station,King Relay,Sverdrup Base,Samos Beacon"
+ },
+ {
+ "name": "Crato",
+ "pos_x": -95,
+ "pos_y": 28.75,
+ "pos_z": 167.5,
+ "stations": "Schiltberger Enterprise,Fast Landing,Cartmill Silo,Quinn Camp"
+ },
+ {
+ "name": "Crescent Sector GW-W c1-8",
+ "pos_x": -4842.1875,
+ "pos_y": 210.8125,
+ "pos_z": 1252.21875,
+ "stations": "Medusa's Rock"
+ },
+ {
+ "name": "Crevit",
+ "pos_x": -24,
+ "pos_y": -85.46875,
+ "pos_z": -1.6875,
+ "stations": "O'Neil One,Betancourt Station,Cummings Bastion"
+ },
+ {
+ "name": "Croatigae",
+ "pos_x": 137.75,
+ "pos_y": -100.375,
+ "pos_z": 25.09375,
+ "stations": "Carrington Hub,Ambartsumian Port,Delaunay Port,Walotsky Point,Fraknoi Ring,Olahus Vision,Qureshi Port,Secchi Port,Cayley Terminal,Barcelona Dock"
+ },
+ {
+ "name": "Crom",
+ "pos_x": 53.0625,
+ "pos_y": -17.34375,
+ "pos_z": 8.46875,
+ "stations": "Chorel Survey"
+ },
+ {
+ "name": "Crom Dubh",
+ "pos_x": 36.6875,
+ "pos_y": -154.875,
+ "pos_z": -2.46875,
+ "stations": "Ball Station,Hornoch Ring,Chiang Beacon,Francis Landing,Jael Relay"
+ },
+ {
+ "name": "Croman",
+ "pos_x": 137.34375,
+ "pos_y": -142.65625,
+ "pos_z": -13.4375,
+ "stations": "van Houten Port,Schwarzschild Dock"
+ },
+ {
+ "name": "Cromen",
+ "pos_x": -102.03125,
+ "pos_y": -58.84375,
+ "pos_z": -46.34375,
+ "stations": "Ivanov Works,Runco Depot"
+ },
+ {
+ "name": "Cromovii",
+ "pos_x": 95.96875,
+ "pos_y": 1.90625,
+ "pos_z": -21.71875,
+ "stations": "Dall Horizons,Desargues Installation,Tshang Dock"
+ },
+ {
+ "name": "Cromovit",
+ "pos_x": 79.84375,
+ "pos_y": -2.5,
+ "pos_z": 25.6875,
+ "stations": "Watts Installation,Guin Dock,Longyear Legacy,Swanwick Prospect,Remek Settlement"
+ },
+ {
+ "name": "Crowfor",
+ "pos_x": 4.625,
+ "pos_y": 20.375,
+ "pos_z": -83,
+ "stations": "Szebehely Port,Vaucanson Market,Makarov Terminal,Apgar City,Simonyi Station,Ewald Station,West Point"
+ },
+ {
+ "name": "Crucis Sector EQ-Y b4",
+ "pos_x": 101,
+ "pos_y": -10.03125,
+ "pos_z": 3.875,
+ "stations": "The Champion of Piety"
+ },
+ {
+ "name": "Crucis Sector GR-V b2-4",
+ "pos_x": 114.6875,
+ "pos_y": 42.90625,
+ "pos_z": 40.125,
+ "stations": "Merchiston Hub,Coblentz Plant"
+ },
+ {
+ "name": "Crucis Sector ND-S b4-5",
+ "pos_x": 78.6875,
+ "pos_y": 53.9375,
+ "pos_z": 80.03125,
+ "stations": "Detention Ship Alpha"
+ },
+ {
+ "name": "Crucis Sector VZ-P b5-5",
+ "pos_x": 84.53125,
+ "pos_y": 9.40625,
+ "pos_z": 101.65625,
+ "stations": "Fozard Silo,Fanning Base"
+ },
+ {
+ "name": "CT Pyxidis",
+ "pos_x": 99.0625,
+ "pos_y": 28.625,
+ "pos_z": -53.1875,
+ "stations": "Stephenson Station,Culbertson Depot"
+ },
+ {
+ "name": "CT Tucanae",
+ "pos_x": 66.40625,
+ "pos_y": -123.375,
+ "pos_z": 51.90625,
+ "stations": "Kurtz Colony,Arantilae,Kapp Port"
+ },
+ {
+ "name": "CU Cancri",
+ "pos_x": 10.5625,
+ "pos_y": 14.5,
+ "pos_z": -22.3125,
+ "stations": "Harvey Platform"
+ },
+ {
+ "name": "Cuachini",
+ "pos_x": -13.21875,
+ "pos_y": 91.15625,
+ "pos_z": 128.34375,
+ "stations": "Gibson Gateway,Eskridge Ring,Lukyanenko Settlement,Goonan's Claim,Davidson's Progress,Shaikh Hub"
+ },
+ {
+ "name": "Cuacocha",
+ "pos_x": -27.6875,
+ "pos_y": -37.6875,
+ "pos_z": 10.90625,
+ "stations": "Bering Hub"
+ },
+ {
+ "name": "Cuages",
+ "pos_x": -137.78125,
+ "pos_y": 21.71875,
+ "pos_z": -14.90625,
+ "stations": "Cartwright Hub,Pullman Keep"
+ },
+ {
+ "name": "Cuagsa",
+ "pos_x": -79.625,
+ "pos_y": -37.84375,
+ "pos_z": 79.59375,
+ "stations": "DeLucas Prospect,Cooper Platform"
+ },
+ {
+ "name": "Cubeo",
+ "pos_x": 128.28125,
+ "pos_y": -155.625,
+ "pos_z": 84.21875,
+ "stations": "Chelomey Orbital,Medupe City,Adelman Station,Weaver Vision,Whittle Orbital,Finch Beacon,Roskam Enterprise,Lubbock Penal colony"
+ },
+ {
+ "name": "Cuberara",
+ "pos_x": -41.21875,
+ "pos_y": -99.6875,
+ "pos_z": 120.75,
+ "stations": "Sabine Camp,Antonio de Andrade Platform"
+ },
+ {
+ "name": "Cubere",
+ "pos_x": -44.71875,
+ "pos_y": -132,
+ "pos_z": 29.1875,
+ "stations": "Mattingly Port,Savery Station,Born Terminal,Schweickart Dock,Bardeen Gateway,White's Folly,Hartlib Installation,Napier's Folly"
+ },
+ {
+ "name": "Cuchaan",
+ "pos_x": -122.15625,
+ "pos_y": -5.8125,
+ "pos_z": 40.84375,
+ "stations": "Bolotov Settlement,Onufriyenko Beacon"
+ },
+ {
+ "name": "Cuchan",
+ "pos_x": 95.375,
+ "pos_y": -72.59375,
+ "pos_z": -84.125,
+ "stations": "Sarmiento de Gamboa Terminal,Cadamosto Orbital,Varley Station,Kress Orbital,Reynolds Enterprise"
+ },
+ {
+ "name": "Cuchoen",
+ "pos_x": 63.625,
+ "pos_y": 15.125,
+ "pos_z": -48.8125,
+ "stations": "Hornblower's Folly,Meikle Settlement"
+ },
+ {
+ "name": "Cuchua",
+ "pos_x": -22.90625,
+ "pos_y": -124.15625,
+ "pos_z": 22.34375,
+ "stations": "Prandtl Dock,Ziegler City,Harrison Hub,Hevelius Station,Kaluta Station,Celsius Installation,Curtis Ring,Hoffman Bastion,Flagg Vision,Lasswitz Terminal,Sucharitkul Plant,Lichtenberg Works,Hulse Prospect"
+ },
+ {
+ "name": "Cuic",
+ "pos_x": 102.09375,
+ "pos_y": 0.53125,
+ "pos_z": -138.125,
+ "stations": "Brady Colony,Hillary Camp"
+ },
+ {
+ "name": "Cuicatec",
+ "pos_x": -101.34375,
+ "pos_y": -141.21875,
+ "pos_z": -39.4375,
+ "stations": "Williamson Orbital,Holub City,Cixin Ring,Nixon Installation,Jones Survey"
+ },
+ {
+ "name": "Cuicaure",
+ "pos_x": -101.59375,
+ "pos_y": -98.9375,
+ "pos_z": -2.34375,
+ "stations": "Korzun Beacon"
+ },
+ {
+ "name": "Cuichit",
+ "pos_x": 37.125,
+ "pos_y": -80.78125,
+ "pos_z": -79.6875,
+ "stations": "McAllaster Laboratory,Herbert City,Moorcock Gateway,Makeev Gateway,Brosnan Base"
+ },
+ {
+ "name": "Cumalam",
+ "pos_x": -20.65625,
+ "pos_y": -73.8125,
+ "pos_z": 128.3125,
+ "stations": "Lambert Prospect,Ambartsumian Dock,Maury Port,Juan de la Cierva Hub,Yamamoto Survey"
+ },
+ {
+ "name": "Cupen",
+ "pos_x": -45.75,
+ "pos_y": 51.25,
+ "pos_z": 80.59375,
+ "stations": "Obruchev Installation,Tilman Arena"
+ },
+ {
+ "name": "Cupera",
+ "pos_x": 32.65625,
+ "pos_y": -204.5625,
+ "pos_z": 129.09375,
+ "stations": "Sagan Relay"
+ },
+ {
+ "name": "Cupiaci",
+ "pos_x": 99.28125,
+ "pos_y": -76.25,
+ "pos_z": 131.5,
+ "stations": "Sandage Station,Poisson Arsenal,Cooper Lab"
+ },
+ {
+ "name": "Cupiat",
+ "pos_x": 28.4375,
+ "pos_y": -6.625,
+ "pos_z": -43,
+ "stations": "Merril Landing,Quimper Orbital,Phillips City"
+ },
+ {
+ "name": "Cupinook",
+ "pos_x": 44.28125,
+ "pos_y": 80.9375,
+ "pos_z": 17.125,
+ "stations": "Russell Gateway,Vonarburg Terminal,Bondar Platform,Malocello City,Moorcock City,Rotsler Terminal,Weyl Terminal,Selous Orbital,Gelfand Lab,Bella Depot,Andrews Dock,Broderick Terminal"
+ },
+ {
+ "name": "Cupis",
+ "pos_x": -72.65625,
+ "pos_y": -16.09375,
+ "pos_z": 56.53125,
+ "stations": "Boodt Port,Brahe Hub,Armstrong Station,Marshall Ring,Kondratyev Dock,Xiaoguan Dock,Stewart Hub,Fairbairn Orbital,Yang's Progress,Poindexter's Claim,Farrukh Port,Silverberg Horizons,Gloss Legacy"
+ },
+ {
+ "name": "Cupisii",
+ "pos_x": 114.96875,
+ "pos_y": -11.03125,
+ "pos_z": -125.5,
+ "stations": "Foale Station,Malenchenko Gateway,Krylov Installation,Houtman Legacy"
+ },
+ {
+ "name": "Curia",
+ "pos_x": 46.46875,
+ "pos_y": -112.53125,
+ "pos_z": 124.8125,
+ "stations": "Juan de la Cierva Landing,Whittle Dock,Bulmer Arsenal"
+ },
+ {
+ "name": "CW Ursae Majoris",
+ "pos_x": 2.65625,
+ "pos_y": 36.78125,
+ "pos_z": -14.90625,
+ "stations": "Fung Settlement,Farouk's Claim,Halley Port,Spring Installation"
+ },
+ {
+ "name": "CX Com",
+ "pos_x": 3.96875,
+ "pos_y": 77.28125,
+ "pos_z": -6.21875,
+ "stations": "Navigator City,Carrasco Orbital,Murphy Relay,Cousin Horizons"
+ },
+ {
+ "name": "Cybele",
+ "pos_x": 33.125,
+ "pos_y": 54.46875,
+ "pos_z": 25.03125,
+ "stations": "Dyomin Ring,Cabrera Orbital,Leslie Orbital,Hornby Port,Teng-hui Hub,Fraley Dock,Morris Installation,Illy Enterprise"
+ },
+ {
+ "name": "Cynetek",
+ "pos_x": 37.28125,
+ "pos_y": 14.3125,
+ "pos_z": 100.625,
+ "stations": "Pudwill Gorie Hub,Morukov Settlement,Barnaby Landing"
+ },
+ {
+ "name": "Cynetes",
+ "pos_x": 156.875,
+ "pos_y": -172.125,
+ "pos_z": -2.75,
+ "stations": "Helin Dock,Moore Vision,Dornier Dock,von Biela Orbital,Nansen's Inheritance"
+ },
+ {
+ "name": "Cyneticels",
+ "pos_x": 20.71875,
+ "pos_y": 125.65625,
+ "pos_z": -61.71875,
+ "stations": "Glenn Gateway,Jakes Beacon,Clark Enterprise"
+ },
+ {
+ "name": "Czerni",
+ "pos_x": 137.09375,
+ "pos_y": -209.21875,
+ "pos_z": 1.09375,
+ "stations": "Ptack Landing,Shajn Relay,Nakano Orbital"
+ },
+ {
+ "name": "Czerno",
+ "pos_x": 111.625,
+ "pos_y": 28.125,
+ "pos_z": -118.3125,
+ "stations": "Yang Colony"
+ },
+ {
+ "name": "Czernobog",
+ "pos_x": -62.65625,
+ "pos_y": -0.6875,
+ "pos_z": -159.1875,
+ "stations": "Bates Dock,Payne Hub"
+ },
+ {
+ "name": "Czernovale",
+ "pos_x": 40.53125,
+ "pos_y": 37.53125,
+ "pos_z": 19.5,
+ "stations": "Savinykh City,Evans Enterprise,Berliner Enterprise,Filter Base"
+ },
+ {
+ "name": "d Scorpii",
+ "pos_x": 24.9375,
+ "pos_y": 34.46875,
+ "pos_z": 127.75,
+ "stations": "Vasquez de Coronado Hub,Wiener Ring,Tapinas Enterprise,Townshend Bastion,Cavendish Relay"
+ },
+ {
+ "name": "Dabakaja",
+ "pos_x": 60.59375,
+ "pos_y": -115.625,
+ "pos_z": -68.875,
+ "stations": "Miyasaka Settlement,Tietjen City,Karachkina Hub,Fife Silo"
+ },
+ {
+ "name": "Dabalngas",
+ "pos_x": -20.03125,
+ "pos_y": 160.6875,
+ "pos_z": -51.21875,
+ "stations": "Landis Mines"
+ },
+ {
+ "name": "Dacian Tse",
+ "pos_x": -30.5,
+ "pos_y": -143.28125,
+ "pos_z": -58.59375,
+ "stations": "Sharman Camp,Beliaev Vision"
+ },
+ {
+ "name": "Dadal",
+ "pos_x": 46.03125,
+ "pos_y": 43.78125,
+ "pos_z": 55.09375,
+ "stations": "Dedman Landing,Fiennes City"
+ },
+ {
+ "name": "Daesi",
+ "pos_x": 86.09375,
+ "pos_y": -117.59375,
+ "pos_z": -56.9375,
+ "stations": "Cherry Station,Zhuravleva Holdings,Connes Vision,Arago Dock,Clayton's Folly"
+ },
+ {
+ "name": "Daesiri",
+ "pos_x": 3.3125,
+ "pos_y": 15.6875,
+ "pos_z": -149.3125,
+ "stations": "Bartlett Platform,Neumann Arena"
+ },
+ {
+ "name": "Daesitiates",
+ "pos_x": 143.46875,
+ "pos_y": 57.09375,
+ "pos_z": 80.90625,
+ "stations": "Ellis Gateway,Blaschke Survey,Stephenson Hub"
+ },
+ {
+ "name": "Daesuratun",
+ "pos_x": 95.9375,
+ "pos_y": -100.84375,
+ "pos_z": 118.28125,
+ "stations": "Becvar Terminal,Struve Mines,Ahern Plant"
+ },
+ {
+ "name": "Dafnharian",
+ "pos_x": 9.1875,
+ "pos_y": -81.75,
+ "pos_z": -115.28125,
+ "stations": "DeLucas Station,Stefansson Camp,Eisele Point"
+ },
+ {
+ "name": "Dafnisto",
+ "pos_x": 102.71875,
+ "pos_y": -152.21875,
+ "pos_z": 69.375,
+ "stations": "Sakers Reach,Seki Platform"
+ },
+ {
+ "name": "Daga tri",
+ "pos_x": -23.1875,
+ "pos_y": -2.625,
+ "pos_z": -100.9375,
+ "stations": "Sverdrup Plant,Grothendieck Terminal,Barbaro Landing"
+ },
+ {
+ "name": "Dagao",
+ "pos_x": 70.15625,
+ "pos_y": -204.53125,
+ "pos_z": 42.25,
+ "stations": "Kelly Port,Flettner Settlement"
+ },
+ {
+ "name": "Dagaz",
+ "pos_x": 30.15625,
+ "pos_y": 55,
+ "pos_z": 15.3125,
+ "stations": "Bohnhoff Hub,Abasheli Landing,Hutton Barracks"
+ },
+ {
+ "name": "Dagdhangjel",
+ "pos_x": -17.8125,
+ "pos_y": -35.78125,
+ "pos_z": 10.84375,
+ "stations": "Low Horizons,Pasteur Terminal,Fischer Base"
+ },
+ {
+ "name": "Dagr",
+ "pos_x": -16.28125,
+ "pos_y": 24.1875,
+ "pos_z": 48.09375,
+ "stations": "Shalatula Colony,Chaviano Platform,Judson Point"
+ },
+ {
+ "name": "Dagudi",
+ "pos_x": -130.9375,
+ "pos_y": 5.65625,
+ "pos_z": 66.84375,
+ "stations": "Wnuk-Lipinski Base,Ricci Survey,Spielberg Platform"
+ },
+ {
+ "name": "Daguteni",
+ "pos_x": 58.25,
+ "pos_y": -24.71875,
+ "pos_z": -147.71875,
+ "stations": "Sawyer Dock"
+ },
+ {
+ "name": "Dagutii",
+ "pos_x": 171.78125,
+ "pos_y": -152.375,
+ "pos_z": 17.375,
+ "stations": "Shaw Horizons,Weill Platform,Ruppelt's Claim"
+ },
+ {
+ "name": "Daha",
+ "pos_x": -1.625,
+ "pos_y": 101.09375,
+ "pos_z": 86.9375,
+ "stations": "Burbank Orbital,Leibniz Station,Bagian Orbital,Tereshkova Hub,Al-Khalili Dock,Mukai Orbital,Tombaugh City,Jun Dock,Asprin Hub,Jacquard City,Lopez de Legazpi Works,Davis Reach,Wellman Survey,Reed Penal colony"
+ },
+ {
+ "name": "Daha Deti",
+ "pos_x": 20.5,
+ "pos_y": -20.90625,
+ "pos_z": 45.78125,
+ "stations": "Carleson Ring,Fremont Silo"
+ },
+ {
+ "name": "Dahaes",
+ "pos_x": 88.90625,
+ "pos_y": -192.59375,
+ "pos_z": 111.21875,
+ "stations": "Kojima Station"
+ },
+ {
+ "name": "Dahambwe",
+ "pos_x": 60.59375,
+ "pos_y": 42,
+ "pos_z": -90.28125,
+ "stations": "Neff Works,Khan Port,Comino Landing"
+ },
+ {
+ "name": "Dahan",
+ "pos_x": -19.75,
+ "pos_y": 41.78125,
+ "pos_z": -3.1875,
+ "stations": "Dahan Gateway,Nelson Works,Dahan 3 Metalworks"
+ },
+ {
+ "name": "Daibais",
+ "pos_x": -19.59375,
+ "pos_y": -103.90625,
+ "pos_z": 144.625,
+ "stations": "Flammarion Terminal,Stein Enterprise,Roche Prospect,Celsius Colony,Ozanne Prospect"
+ },
+ {
+ "name": "Daibamba",
+ "pos_x": -116.65625,
+ "pos_y": -15.9375,
+ "pos_z": -62.3125,
+ "stations": "Kovalyonok Enterprise"
+ },
+ {
+ "name": "Daibara",
+ "pos_x": -102.5625,
+ "pos_y": 76.46875,
+ "pos_z": -41.375,
+ "stations": "McKay Port,Palmer Hub,Rusch Orbital,Sabine Enterprise"
+ },
+ {
+ "name": "Daibo",
+ "pos_x": 165.96875,
+ "pos_y": -169.28125,
+ "pos_z": 101.3125,
+ "stations": "Brundage Dock,Maddox Barracks"
+ },
+ {
+ "name": "Daiburi",
+ "pos_x": 125.1875,
+ "pos_y": -14.71875,
+ "pos_z": 48.46875,
+ "stations": "Bakewell Installation,Tsibliyev Station"
+ },
+ {
+ "name": "Daibus",
+ "pos_x": 117.90625,
+ "pos_y": 111.65625,
+ "pos_z": 36.1875,
+ "stations": "Fossum Works,Forest Landing,Snodgrass' Inheritance"
+ },
+ {
+ "name": "Daii",
+ "pos_x": 142.71875,
+ "pos_y": -45.15625,
+ "pos_z": 111.6875,
+ "stations": "Sopwith Enterprise,Levinson Prospect,Speke Port,Mohr Mines,Giclas Hub"
+ },
+ {
+ "name": "Daik",
+ "pos_x": -69.0625,
+ "pos_y": -22.375,
+ "pos_z": -148.09375,
+ "stations": "Bulmer Outpost,Gottlob Frege Vision"
+ },
+ {
+ "name": "Daikonjungu",
+ "pos_x": 139.4375,
+ "pos_y": -63.90625,
+ "pos_z": -53.125,
+ "stations": "Shepard Oasis,Comer Works,Moorcock Mines,Lasswitz Installation"
+ },
+ {
+ "name": "Daiku",
+ "pos_x": 24.96875,
+ "pos_y": 2.84375,
+ "pos_z": 29.03125,
+ "stations": "Chaviano Station,Scheutz Keep,Pinto Point,Wrangel Orbital,Levi-Montalcini Beacon"
+ },
+ {
+ "name": "Daikul",
+ "pos_x": 114.0625,
+ "pos_y": 106.78125,
+ "pos_z": -104.15625,
+ "stations": "Denton's Inheritance"
+ },
+ {
+ "name": "Daikulcandi",
+ "pos_x": 57.40625,
+ "pos_y": -118.4375,
+ "pos_z": 50.84375,
+ "stations": "Chretien Oudemans Hangar,Fraunhofer Installation,Krenkel Station"
+ },
+ {
+ "name": "Dain",
+ "pos_x": -43.8125,
+ "pos_y": -67.5,
+ "pos_z": 91.53125,
+ "stations": "Gottlob Frege Mine"
+ },
+ {
+ "name": "Daina",
+ "pos_x": 89.375,
+ "pos_y": 31.28125,
+ "pos_z": 138.9375,
+ "stations": "Block Landing,Heceta City"
+ },
+ {
+ "name": "Dainayiman",
+ "pos_x": 144.5625,
+ "pos_y": -104.6875,
+ "pos_z": 28.46875,
+ "stations": "Cheranovsky Gateway,Smyth Ring,Biggle Relay,Verne Enterprise"
+ },
+ {
+ "name": "Dainbaras",
+ "pos_x": 162.78125,
+ "pos_y": 46.9375,
+ "pos_z": -73.25,
+ "stations": "Huberath Port"
+ },
+ {
+ "name": "Dainbing",
+ "pos_x": -58.875,
+ "pos_y": -96.9375,
+ "pos_z": -71.3125,
+ "stations": "Niven Landing,Russell Mine"
+ },
+ {
+ "name": "Daini",
+ "pos_x": 137.59375,
+ "pos_y": -17.03125,
+ "pos_z": -55.96875,
+ "stations": "Ford Dock"
+ },
+ {
+ "name": "Dais",
+ "pos_x": 100.4375,
+ "pos_y": -204.65625,
+ "pos_z": 28.21875,
+ "stations": "Elmore Escape"
+ },
+ {
+ "name": "Daisamaskap",
+ "pos_x": -136.125,
+ "pos_y": 90.71875,
+ "pos_z": 32.34375,
+ "stations": "Krusvar Dock,Andrews Penal colony,Francisco de Almeida Freeport,Froude Dock"
+ },
+ {
+ "name": "Daisina",
+ "pos_x": 78.625,
+ "pos_y": -53.125,
+ "pos_z": -100.46875,
+ "stations": "Stephenson's Claim,Godwin Asylum,Galiano Survey"
+ },
+ {
+ "name": "Daisla",
+ "pos_x": 136.15625,
+ "pos_y": 3.4375,
+ "pos_z": -82.84375,
+ "stations": "Darwin Legacy,Musabayev Beacon"
+ },
+ {
+ "name": "Daiti",
+ "pos_x": 55.5,
+ "pos_y": 9.875,
+ "pos_z": -42.53125,
+ "stations": "Beckman Market,Edgeworth Gateway,Remek Hub"
+ },
+ {
+ "name": "Dajoar",
+ "pos_x": -35.0625,
+ "pos_y": 39.1875,
+ "pos_z": 92.78125,
+ "stations": "Crown Terminal,Thiele Port,Patrick Terminal,Ejeta Enterprise,Cochrane Horizons,Kiernan Camp,Macquorn Rankine Port,Shiras Silo"
+ },
+ {
+ "name": "Dajoarama",
+ "pos_x": 77.65625,
+ "pos_y": -44.25,
+ "pos_z": 86.34375,
+ "stations": "Bolton Dock"
+ },
+ {
+ "name": "Dakak",
+ "pos_x": 124.75,
+ "pos_y": -141.75,
+ "pos_z": 120.9375,
+ "stations": "Orbik Station,Youll Colony,Kuchner Prospect"
+ },
+ {
+ "name": "Dakatipini",
+ "pos_x": 26.78125,
+ "pos_y": -177.65625,
+ "pos_z": 132.9375,
+ "stations": "Jefferies Dock,Williamson Silo"
+ },
+ {
+ "name": "Dakinn",
+ "pos_x": -140.40625,
+ "pos_y": -24.15625,
+ "pos_z": -59.09375,
+ "stations": "Kovalyonok Enterprise"
+ },
+ {
+ "name": "Dakondunii",
+ "pos_x": -87.5625,
+ "pos_y": 12.4375,
+ "pos_z": -141.4375,
+ "stations": "Asire Arena,Robinson Installation"
+ },
+ {
+ "name": "Daksakhi",
+ "pos_x": 89.46875,
+ "pos_y": 58.5625,
+ "pos_z": 39.65625,
+ "stations": "Hoften Terminal,Morgan Terminal,Nasmyth City,Teller Dock,Dalton Enterprise,Boming Gateway,Diophantus Market,Baker Port,Potagos Barracks,Lavrador Holdings"
+ },
+ {
+ "name": "Dakshalli",
+ "pos_x": 121.21875,
+ "pos_y": -56.6875,
+ "pos_z": 24.3125,
+ "stations": "Hereford Vision,Reeves Dock,Minkowski Camp,Martinez Barracks"
+ },
+ {
+ "name": "Dakshey",
+ "pos_x": -91.6875,
+ "pos_y": -51.25,
+ "pos_z": -95.34375,
+ "stations": "Goeppert-Mayer Mine"
+ },
+ {
+ "name": "Dakshmandi",
+ "pos_x": 106.21875,
+ "pos_y": -127.65625,
+ "pos_z": 37.65625,
+ "stations": "Hevelius Enterprise,Kibalchich Enterprise,Foglio Hub"
+ },
+ {
+ "name": "Dakshu",
+ "pos_x": -39.96875,
+ "pos_y": 49.09375,
+ "pos_z": 79.40625,
+ "stations": "Rubruck Dock,Lefschetz Base"
+ },
+ {
+ "name": "Dakvar",
+ "pos_x": 5.96875,
+ "pos_y": -81.4375,
+ "pos_z": -31.71875,
+ "stations": "Port Bueschel,Naglotech"
+ },
+ {
+ "name": "Dala",
+ "pos_x": 24.75,
+ "pos_y": 25.90625,
+ "pos_z": -22.0625,
+ "stations": "Fincke Platform,Gidzenko Base,Gillekens Landing"
+ },
+ {
+ "name": "Dalagritu",
+ "pos_x": -75.46875,
+ "pos_y": 178.15625,
+ "pos_z": -58.375,
+ "stations": "Quimper Gateway,Spielberg Ring,Davidson Hub,Moorcock Relay"
+ },
+ {
+ "name": "Dalam",
+ "pos_x": 83.59375,
+ "pos_y": 14.71875,
+ "pos_z": 3.53125,
+ "stations": "Bunsen Bastion,Kraepelin Hangar"
+ },
+ {
+ "name": "Dalang",
+ "pos_x": -40.9375,
+ "pos_y": 0,
+ "pos_z": 162.84375,
+ "stations": "Mouhot Survey,Norman Colony,Scithers Holdings"
+ },
+ {
+ "name": "Dalannovale",
+ "pos_x": 98.875,
+ "pos_y": 6.71875,
+ "pos_z": -34.40625,
+ "stations": "Abernathy Relay,Siodmak Prospect"
+ },
+ {
+ "name": "Dalat",
+ "pos_x": 196.65625,
+ "pos_y": -159.15625,
+ "pos_z": 4.71875,
+ "stations": "Schuster Terminal,Beliaev Survey"
+ },
+ {
+ "name": "Dalevard",
+ "pos_x": -47,
+ "pos_y": 36.6875,
+ "pos_z": -62.375,
+ "stations": "Shepherd City,Sharman Hub,Haxel City,Cartwright Installation"
+ },
+ {
+ "name": "Dalfur",
+ "pos_x": -11.09375,
+ "pos_y": -40.34375,
+ "pos_z": -73.0625,
+ "stations": "Peirce Landing,Aquila Station,Nansen Enterprise,Bering Mines,Whymper Works"
+ },
+ {
+ "name": "Dalia",
+ "pos_x": -2.65625,
+ "pos_y": -58.28125,
+ "pos_z": 16.34375,
+ "stations": "McCormick Penal colony,MacKellar Horizons"
+ },
+ {
+ "name": "Dall",
+ "pos_x": 73.15625,
+ "pos_y": -143.90625,
+ "pos_z": 37.4375,
+ "stations": "Howe Platform,Wolszczan Beacon,Parker Platform"
+ },
+ {
+ "name": "Dalla",
+ "pos_x": 33.0625,
+ "pos_y": 67.8125,
+ "pos_z": -27.6875,
+ "stations": "Mitchell Gateway,Crown Bastion,Gorbatko City,Debye City"
+ },
+ {
+ "name": "Dallean",
+ "pos_x": -148.28125,
+ "pos_y": 38.46875,
+ "pos_z": 34.09375,
+ "stations": "Thompson Dock,Kozin Base"
+ },
+ {
+ "name": "Dallenja",
+ "pos_x": -121.65625,
+ "pos_y": -36.9375,
+ "pos_z": -34.46875,
+ "stations": "Bresnik Retreat,Toll's Progress,Pennington Port"
+ },
+ {
+ "name": "Damna",
+ "pos_x": -40.53125,
+ "pos_y": 4.375,
+ "pos_z": -124.25,
+ "stations": "Nemere Market,Clute Orbital,Nordenskiold Port,Popper Enterprise,Gilliland Dock,Bao City,Maybury Survey,Egan Port"
+ },
+ {
+ "name": "Damnet",
+ "pos_x": 55.40625,
+ "pos_y": -43.40625,
+ "pos_z": 75.5625,
+ "stations": "Acton Relay"
+ },
+ {
+ "name": "Damnon",
+ "pos_x": -28.78125,
+ "pos_y": 148.0625,
+ "pos_z": -37.1875,
+ "stations": "Hamilton Station,Yu Orbital,Staden Terminal,Alexander Installation"
+ },
+ {
+ "name": "Damocan",
+ "pos_x": -13.25,
+ "pos_y": -29.71875,
+ "pos_z": 75.125,
+ "stations": "Chadwick Terminal,Cousteau Horizons,Smith Silo"
+ },
+ {
+ "name": "Damoch",
+ "pos_x": 78.75,
+ "pos_y": -26.4375,
+ "pos_z": 87.25,
+ "stations": "Burns Retreat"
+ },
+ {
+ "name": "Damona",
+ "pos_x": 78.0625,
+ "pos_y": -141.46875,
+ "pos_z": 61.09375,
+ "stations": "Littrow City,Merle Lab,Narlikar Base"
+ },
+ {
+ "name": "Damontae",
+ "pos_x": 134.0625,
+ "pos_y": -35.09375,
+ "pos_z": -8.96875,
+ "stations": "Albategnius City"
+ },
+ {
+ "name": "Damoorai",
+ "pos_x": 163.53125,
+ "pos_y": -167.625,
+ "pos_z": -12.875,
+ "stations": "Allen St. John Station,Oxley Laboratory,Lee Arsenal"
+ },
+ {
+ "name": "Damovik",
+ "pos_x": -122.125,
+ "pos_y": -127.09375,
+ "pos_z": 15.375,
+ "stations": "Shepherd Port"
+ },
+ {
+ "name": "Dan",
+ "pos_x": -19.375,
+ "pos_y": -101.84375,
+ "pos_z": -141.34375,
+ "stations": "Haldeman II Terminal,Gunn Port,Payne Dock"
+ },
+ {
+ "name": "Dana",
+ "pos_x": 46.09375,
+ "pos_y": -192.625,
+ "pos_z": 24.15625,
+ "stations": "Phillips' Pride,Nouvel Sanctuary,Faber Hub"
+ },
+ {
+ "name": "Dang Po",
+ "pos_x": -61.3125,
+ "pos_y": 30.28125,
+ "pos_z": -144.75,
+ "stations": "Bridger Vision"
+ },
+ {
+ "name": "Dangai",
+ "pos_x": -28.78125,
+ "pos_y": -9.625,
+ "pos_z": 126.15625,
+ "stations": "Bessemer Station,Gernhardt Horizons,Caillie Prospect,Oswald Barracks"
+ },
+ {
+ "name": "Dangarla",
+ "pos_x": 59.28125,
+ "pos_y": -42.125,
+ "pos_z": -26.59375,
+ "stations": "Swanson Enterprise"
+ },
+ {
+ "name": "Danggali",
+ "pos_x": -14.03125,
+ "pos_y": -76.71875,
+ "pos_z": 131.25,
+ "stations": "Soukup Hub,Savorgnan de Brazza Hub"
+ },
+ {
+ "name": "Dangkhurs",
+ "pos_x": 118.34375,
+ "pos_y": -222.84375,
+ "pos_z": -21.125,
+ "stations": "Altuna Stop"
+ },
+ {
+ "name": "Dango",
+ "pos_x": -19.53125,
+ "pos_y": -140.375,
+ "pos_z": 35.46875,
+ "stations": "Bokeili Enterprise,Abbot Relay"
+ },
+ {
+ "name": "Dangtei",
+ "pos_x": 146.8125,
+ "pos_y": -151.4375,
+ "pos_z": 23.78125,
+ "stations": "Harrington Station,Kotzebue Enterprise,Ilyushin Enterprise,Siegel Terminal"
+ },
+ {
+ "name": "Dangu",
+ "pos_x": 4.3125,
+ "pos_y": -168.46875,
+ "pos_z": -16.71875,
+ "stations": "Gabrielli Hub,Messier Works,Naubakht Survey"
+ },
+ {
+ "name": "Dao",
+ "pos_x": -17.375,
+ "pos_y": 131.25,
+ "pos_z": 83.9375,
+ "stations": "Tenn Sanctuary"
+ },
+ {
+ "name": "Dao Guaras",
+ "pos_x": -91,
+ "pos_y": 89.53125,
+ "pos_z": 67.40625,
+ "stations": "Titov Relay,Wandrei Holdings"
+ },
+ {
+ "name": "Dao Jungu",
+ "pos_x": 15.78125,
+ "pos_y": 35.34375,
+ "pos_z": 65.21875,
+ "stations": "Landis Terminal,Carlisle Orbital,Dayuan Orbital,Swanwick Port,Mille City,Kessel Gateway,Ron Hubbard Hub,Gresh Installation,Vercors Orbital,Bayley Station,Kimberlin Dock,Grigson Survey,Fairbairn Gateway"
+ },
+ {
+ "name": "Dao Kach",
+ "pos_x": 56.375,
+ "pos_y": 97.96875,
+ "pos_z": 11,
+ "stations": "Miletus Terminal,Pontes Refinery,Sherrington Landing"
+ },
+ {
+ "name": "Dao Tiensi",
+ "pos_x": -69.125,
+ "pos_y": -179.5625,
+ "pos_z": 39.3125,
+ "stations": "Drake's Progress"
+ },
+ {
+ "name": "Dao Tzu",
+ "pos_x": -36.65625,
+ "pos_y": -124.28125,
+ "pos_z": -99.1875,
+ "stations": "Coelho Terminal"
+ },
+ {
+ "name": "Dao Zi",
+ "pos_x": 12.875,
+ "pos_y": 114.375,
+ "pos_z": 29,
+ "stations": "Savitskaya Gateway,Bohr Gateway,Coleman Orbital"
+ },
+ {
+ "name": "Daori",
+ "pos_x": -61.96875,
+ "pos_y": 115.25,
+ "pos_z": 16.53125,
+ "stations": "Benford Installation,Clement Depot"
+ },
+ {
+ "name": "Darahk",
+ "pos_x": -65,
+ "pos_y": 27,
+ "pos_z": -37,
+ "stations": "Noblehome,Karl Diesel's Folly"
+ },
+ {
+ "name": "Daraj",
+ "pos_x": 81.65625,
+ "pos_y": -103.5625,
+ "pos_z": 99.78125,
+ "stations": "Froud Hub,Biggle Penal colony,Bokeili Landing"
+ },
+ {
+ "name": "Daraki",
+ "pos_x": 22.65625,
+ "pos_y": 129.21875,
+ "pos_z": -6.09375,
+ "stations": "Drexler Gateway,Tanner Enterprise,Lorenz Hub,Nielsen Palace,McAuley Vision"
+ },
+ {
+ "name": "Daralas",
+ "pos_x": 76.5625,
+ "pos_y": -160.125,
+ "pos_z": 100.1875,
+ "stations": "Mozhaysky Colony,Carrington Base"
+ },
+ {
+ "name": "Daramo",
+ "pos_x": -5.375,
+ "pos_y": -74,
+ "pos_z": 21.9375,
+ "stations": "Gregory Enterprise,Birkeland Dock,Knight Port,Atwater Enterprise,Selye Enterprise,Samokutyayev Station,Daimler Dock,Archimedes Enterprise,Broglie Relay,Pasteur Ring,Palmer Point,Ellison Observatory"
+ },
+ {
+ "name": "Daras",
+ "pos_x": -107.6875,
+ "pos_y": 82.78125,
+ "pos_z": -21.75,
+ "stations": "Szentmartony Port,Barton Dock,Maybury Base,Greenleaf Beacon"
+ },
+ {
+ "name": "Dari",
+ "pos_x": 14.4375,
+ "pos_y": -192.53125,
+ "pos_z": 123.375,
+ "stations": "Kandrup Mines"
+ },
+ {
+ "name": "Darian",
+ "pos_x": -88.46875,
+ "pos_y": -16.90625,
+ "pos_z": 95.40625,
+ "stations": "Palmer Works,Wedge Camp,Pogue Landing"
+ },
+ {
+ "name": "Daris",
+ "pos_x": -63.78125,
+ "pos_y": 88.28125,
+ "pos_z": 66.96875,
+ "stations": "Gagnan Colony,Meitner Refinery,Tavernier Observatory"
+ },
+ {
+ "name": "Darkan",
+ "pos_x": -120,
+ "pos_y": -72.78125,
+ "pos_z": 35.4375,
+ "stations": "Lee Platform,DeLucas Landing"
+ },
+ {
+ "name": "Darkates",
+ "pos_x": 96.96875,
+ "pos_y": -164.40625,
+ "pos_z": 137.40625,
+ "stations": "Suzuki Relay"
+ },
+ {
+ "name": "Darkinu",
+ "pos_x": -99.875,
+ "pos_y": -23.75,
+ "pos_z": -120.6875,
+ "stations": "Vinge Platform,Davidson Landing"
+ },
+ {
+ "name": "Darku",
+ "pos_x": 13.21875,
+ "pos_y": 24.375,
+ "pos_z": 142.90625,
+ "stations": "H. G. Wells Landing,Baker City,Malocello Landing"
+ },
+ {
+ "name": "Darudnikepa",
+ "pos_x": -99.15625,
+ "pos_y": -6.1875,
+ "pos_z": -20.25,
+ "stations": "Xuanzang Ring"
+ },
+ {
+ "name": "Daruwach",
+ "pos_x": -109.875,
+ "pos_y": 45.46875,
+ "pos_z": -73.84375,
+ "stations": "Nearchus Hub,Derleth Settlement,Sawyer Prospect"
+ },
+ {
+ "name": "Daruwutja",
+ "pos_x": -81.75,
+ "pos_y": -48.53125,
+ "pos_z": -62.4375,
+ "stations": "Green Estate,Emshwiller Vision,Bering Bastion"
+ },
+ {
+ "name": "Dasar",
+ "pos_x": 111.84375,
+ "pos_y": -120.25,
+ "pos_z": 49.0625,
+ "stations": "Freas Beacon,Lyot Vision,Luther Stop"
+ },
+ {
+ "name": "Dassapic",
+ "pos_x": 79.9375,
+ "pos_y": 113.3125,
+ "pos_z": -3.21875,
+ "stations": "Aguirre Mine"
+ },
+ {
+ "name": "Dassareti",
+ "pos_x": -106.84375,
+ "pos_y": 22.4375,
+ "pos_z": -1.625,
+ "stations": "Pailes Station,Feoktistov Hub,Haignere Enterprise,Maudslay Colony"
+ },
+ {
+ "name": "Dassi",
+ "pos_x": 187.53125,
+ "pos_y": -121.25,
+ "pos_z": 36.0625,
+ "stations": "Gustav Sporer Survey"
+ },
+ {
+ "name": "Dasyapor",
+ "pos_x": 70.6875,
+ "pos_y": 151.6875,
+ "pos_z": 69.3125,
+ "stations": "Dietz Settlement,Zebrowski Colony,Carr Freeport,Binder Barracks,Beltrami Horizons"
+ },
+ {
+ "name": "Daurtu",
+ "pos_x": -47.53125,
+ "pos_y": 48.90625,
+ "pos_z": -110.90625,
+ "stations": "Panshin Terminal"
+ },
+ {
+ "name": "Dauruma",
+ "pos_x": 54.15625,
+ "pos_y": -212.4375,
+ "pos_z": -6.8125,
+ "stations": "Auwers Barracks,Hayashi Port"
+ },
+ {
+ "name": "Dazhbogati",
+ "pos_x": -120.21875,
+ "pos_y": -41.09375,
+ "pos_z": -17.53125,
+ "stations": "Lawson Terminal,Barsanti Station,Lousma City"
+ },
+ {
+ "name": "Dea Motrona",
+ "pos_x": -12.15625,
+ "pos_y": 62.625,
+ "pos_z": 29.71875,
+ "stations": "Pinzon Dock,Scalzi Beacon,Samos Depot"
+ },
+ {
+ "name": "Deacon's Star",
+ "pos_x": 39.1875,
+ "pos_y": -55.0625,
+ "pos_z": 58.375,
+ "stations": "Mouchez Platform"
+ },
+ {
+ "name": "Decamandak",
+ "pos_x": -138.9375,
+ "pos_y": 26.46875,
+ "pos_z": 112.125,
+ "stations": "Griffith Mines,d'Eyncourt Enterprise,Offutt Base"
+ },
+ {
+ "name": "Decan",
+ "pos_x": -0.625,
+ "pos_y": -5.21875,
+ "pos_z": 134.28125,
+ "stations": "Jones Escape"
+ },
+ {
+ "name": "Decantae",
+ "pos_x": 145.34375,
+ "pos_y": -123.4375,
+ "pos_z": 76.4375,
+ "stations": "Harrington Port,Oja Park,Dubyago Platform"
+ },
+ {
+ "name": "Decean",
+ "pos_x": 171.375,
+ "pos_y": -17.65625,
+ "pos_z": 61.34375,
+ "stations": "Levi-Civita Port,Cramer Depot,Fincke Terminal"
+ },
+ {
+ "name": "Deceangli",
+ "pos_x": 11.65625,
+ "pos_y": 12.65625,
+ "pos_z": 99.78125,
+ "stations": "Coulomb Horizons,Savorgnan de Brazza City,Reynolds Hub,Adams Lab"
+ },
+ {
+ "name": "Dech",
+ "pos_x": 154.25,
+ "pos_y": 27.65625,
+ "pos_z": 58.5,
+ "stations": "Lyell Landing,Griffith's Folly"
+ },
+ {
+ "name": "Deche",
+ "pos_x": -170.9375,
+ "pos_y": 2.59375,
+ "pos_z": 80.1875,
+ "stations": "Rusch Prospect,Hodgson Hub,Drummond Holdings"
+ },
+ {
+ "name": "Dechengh",
+ "pos_x": 96.8125,
+ "pos_y": -23,
+ "pos_z": -94.4375,
+ "stations": "Obruchev Depot,Fischer City,Gibbs Landing,Ray Orbital"
+ },
+ {
+ "name": "Decht",
+ "pos_x": 20.125,
+ "pos_y": -7.96875,
+ "pos_z": 156.90625,
+ "stations": "Cauchy Dock"
+ },
+ {
+ "name": "Deciani",
+ "pos_x": 46.59375,
+ "pos_y": -57.09375,
+ "pos_z": 72.5625,
+ "stations": "Dunlop's Pride"
+ },
+ {
+ "name": "Deciat",
+ "pos_x": 122.625,
+ "pos_y": -0.8125,
+ "pos_z": -47.28125,
+ "stations": "Farseer Inc,Garay Terminal,Kirtley Vision,Carson Hub,Matteucci Dock,Hasse Point"
+ },
+ {
+ "name": "Decima",
+ "pos_x": 35.9375,
+ "pos_y": -34.4375,
+ "pos_z": -30.4375,
+ "stations": "Shakoor's Stand,Gustypants Outback Emporium,Wisniewski-Snerg Relay,Rucker Depot"
+ },
+ {
+ "name": "Decis",
+ "pos_x": -82.5625,
+ "pos_y": -64.875,
+ "pos_z": 61.59375,
+ "stations": "Nourse Legacy"
+ },
+ {
+ "name": "Dedwender",
+ "pos_x": 15.375,
+ "pos_y": 57.3125,
+ "pos_z": -122.3125,
+ "stations": "Hovgaard Installation,Jemison Station"
+ },
+ {
+ "name": "Degardhi",
+ "pos_x": 33.65625,
+ "pos_y": -205.71875,
+ "pos_z": -37.6875,
+ "stations": "Joy Landing,Hickam Holdings,Mukai Enterprise"
+ },
+ {
+ "name": "Degastani",
+ "pos_x": -118.5625,
+ "pos_y": -75.5625,
+ "pos_z": 26.78125,
+ "stations": "Francisco de Eliza's Inheritance,Weiss Orbital,Sargent Gateway"
+ },
+ {
+ "name": "Deili",
+ "pos_x": -20.3125,
+ "pos_y": 73.59375,
+ "pos_z": 62.46875,
+ "stations": "McArthur Works"
+ },
+ {
+ "name": "Deiva",
+ "pos_x": 104.4375,
+ "pos_y": 73.5625,
+ "pos_z": 82.3125,
+ "stations": "O'Leary Ring,Herreshoff Orbital,Lindbohm Port,Alvares Depot,Scott Mines"
+ },
+ {
+ "name": "Deive",
+ "pos_x": -121.25,
+ "pos_y": -99.40625,
+ "pos_z": 9.1875,
+ "stations": "Obruchev Orbital,Soto Enterprise,Nikitin Vision"
+ },
+ {
+ "name": "Delkar",
+ "pos_x": 67.8125,
+ "pos_y": 32.65625,
+ "pos_z": 35.25,
+ "stations": "Caillie Landing,de Kamp Orbital,Invergary"
+ },
+ {
+ "name": "Delphin",
+ "pos_x": 18.65625,
+ "pos_y": 16.75,
+ "pos_z": -76.3125,
+ "stations": "Aristotle Orbital,Kondratyev Orbital,Swigert Orbital,Smith Base,Joliot-Curie Dock,Clark Orbital,Grant's Inheritance,Thomas Depot"
+ },
+ {
+ "name": "Delta Doradus",
+ "pos_x": 127.15625,
+ "pos_y": -77.875,
+ "pos_z": 12.125,
+ "stations": "Tousey Dock,Terry Survey,Vaucouleurs Bastion"
+ },
+ {
+ "name": "Delta Equulei",
+ "pos_x": -47.4375,
+ "pos_y": -26.46875,
+ "pos_z": 26.1875,
+ "stations": "Bisson Hub,Moriarty Horizons"
+ },
+ {
+ "name": "Delta Horologii",
+ "pos_x": 112.21875,
+ "pos_y": -130.1875,
+ "pos_z": -49.3125,
+ "stations": "Ashbrook Dock,Lagadha Colony"
+ },
+ {
+ "name": "Delta Leporis",
+ "pos_x": 76,
+ "pos_y": -42.15625,
+ "pos_z": -73.34375,
+ "stations": "Vardeman Orbital,McMullen Hub,Biggle Port,Soukup Terminal,Due Station,Robson Dock,Corte-Real Station"
+ },
+ {
+ "name": "Delta Muscae",
+ "pos_x": 74.375,
+ "pos_y": -14.1875,
+ "pos_z": 50.28125,
+ "stations": "Poleshchuk Station,Andreas Ring,Mille Relay,Lyakhov Terminal,Bates Relay,Oefelein Terminal,Ford Ring,Malpighi Point,Swainson Enterprise"
+ },
+ {
+ "name": "Delta Normae",
+ "pos_x": 50.625,
+ "pos_y": 9.90625,
+ "pos_z": 110.90625,
+ "stations": "Ferguson Relay,Good Dock"
+ },
+ {
+ "name": "Delta Pavonis",
+ "pos_x": 8.375,
+ "pos_y": -10.84375,
+ "pos_z": 14.46875,
+ "stations": "Hooper Relay,Schneider Orbiter,Stephenson Orbital,Deere Holdings"
+ },
+ {
+ "name": "Delta Phoenicis",
+ "pos_x": 53.90625,
+ "pos_y": -130.6875,
+ "pos_z": 14.65625,
+ "stations": "Trading Post,Sandage Terminal,Svahn's Trading Station"
+ },
+ {
+ "name": "Delta-2 Canis Minoris",
+ "pos_x": 76.90625,
+ "pos_y": 26.71875,
+ "pos_z": -109.1875,
+ "stations": "Atwater Station,McDivitt City,Blaylock Survey,Lanchester Escape"
+ },
+ {
+ "name": "Demeter",
+ "pos_x": -55.34375,
+ "pos_y": 40.8125,
+ "pos_z": 7.5625,
+ "stations": "Young Ring,Heinlein Ring"
+ },
+ {
+ "name": "Demeti",
+ "pos_x": 83.28125,
+ "pos_y": -51.125,
+ "pos_z": 102.03125,
+ "stations": "Malchiodi Ring"
+ },
+ {
+ "name": "Deneb Algedi",
+ "pos_x": -16.5,
+ "pos_y": -28.09375,
+ "pos_z": 20.875,
+ "stations": "Burke Port,Conti Penal colony,Fadlan Refinery,Fraser Refinery,Leckie Holdings"
+ },
+ {
+ "name": "Deneb el Okab",
+ "pos_x": -114.09375,
+ "pos_y": 12.375,
+ "pos_z": 104.125,
+ "stations": "Pelliot Depot,Merle Installation"
+ },
+ {
+ "name": "Deneb Okab",
+ "pos_x": -134.71875,
+ "pos_y": -32.84375,
+ "pos_z": 120.28125,
+ "stations": "Gallun Landing,Carlisle Relay"
+ },
+ {
+ "name": "Denebola",
+ "pos_x": 11.09375,
+ "pos_y": 33.90625,
+ "pos_z": -3.5,
+ "stations": "Dirac Terminal,O'Donnell Barracks"
+ },
+ {
+ "name": "Denka",
+ "pos_x": 74.0625,
+ "pos_y": -138.59375,
+ "pos_z": 33.9375,
+ "stations": "Gernsback Vision,Beriev Colony,Cesar Janssen Survey,Backlund Prospect"
+ },
+ {
+ "name": "Deorii",
+ "pos_x": 175.875,
+ "pos_y": -136.75,
+ "pos_z": 42.40625,
+ "stations": "Zhukovsky City,Giraud Vision,Jones Orbital,Resnik Works,Melia Works"
+ },
+ {
+ "name": "Deorini",
+ "pos_x": 154.5625,
+ "pos_y": -69.78125,
+ "pos_z": -29.90625,
+ "stations": "William Sargent Enterprise,Collins Depot"
+ },
+ {
+ "name": "Deorinik",
+ "pos_x": -86.3125,
+ "pos_y": -18.25,
+ "pos_z": 62.53125,
+ "stations": "Lee Hub,Hodgkin Prospect,Sohl Works"
+ },
+ {
+ "name": "Deoriorii",
+ "pos_x": 193.4375,
+ "pos_y": -119.09375,
+ "pos_z": 18.34375,
+ "stations": "Goryu Settlement"
+ },
+ {
+ "name": "Derebititja",
+ "pos_x": -108.1875,
+ "pos_y": -89.15625,
+ "pos_z": -41.625,
+ "stations": "Abasheli Vision,Heceta Depot,Rey's Folly"
+ },
+ {
+ "name": "Deriso",
+ "pos_x": -9520.3125,
+ "pos_y": -909.5,
+ "pos_z": 19808.75,
+ "stations": "Giles Station,Talalay Retreat"
+ },
+ {
+ "name": "Deriv-Dar",
+ "pos_x": -4.46875,
+ "pos_y": 11,
+ "pos_z": -82.71875,
+ "stations": "Hertz Installation,Siemens Station,Duckworth City,Yaping Port,Kekule City,Davy Terminal,Thomas Gateway,Sharman Orbital,Nespoli Orbital,Stillman Settlement,Oluwafemi Base,Einstein Keep"
+ },
+ {
+ "name": "Derrim",
+ "pos_x": -16.625,
+ "pos_y": -32.40625,
+ "pos_z": 81,
+ "stations": "Nachtigal Hub,Regiomontanus Hub,Miletus Enterprise,Rae Keep"
+ },
+ {
+ "name": "Desuanta",
+ "pos_x": -36.90625,
+ "pos_y": -110.65625,
+ "pos_z": -80.6875,
+ "stations": "Thomson Survey,Ewald Dock"
+ },
+ {
+ "name": "Desubi",
+ "pos_x": 63.625,
+ "pos_y": -80.90625,
+ "pos_z": 159.0625,
+ "stations": "Lloyd Wright Terminal,Hay Hub,Baade Orbital,Poncelet Market,Alexander Enterprise"
+ },
+ {
+ "name": "Desurinbin",
+ "pos_x": 140.5,
+ "pos_y": 28.1875,
+ "pos_z": -117.0625,
+ "stations": "Maury Dock,Clair Gateway,Tudela Prospect,Spinrad Hub,Spielberg Ring"
+ },
+ {
+ "name": "Desy",
+ "pos_x": -9534.21875,
+ "pos_y": -912.21875,
+ "pos_z": 19792.375,
+ "stations": "Morten's Paradise"
+ },
+ {
+ "name": "Detris",
+ "pos_x": 67.71875,
+ "pos_y": -57.40625,
+ "pos_z": -8.59375,
+ "stations": "Rogers Orbital"
+ },
+ {
+ "name": "Detta",
+ "pos_x": -40.125,
+ "pos_y": 6.28125,
+ "pos_z": 92.03125,
+ "stations": "Schwann Orbital,Lister Station,Ford Gateway,Kononenko Port,Low Gateway"
+ },
+ {
+ "name": "Deurber",
+ "pos_x": 11.40625,
+ "pos_y": 40.71875,
+ "pos_z": 130.28125,
+ "stations": "Harrison Settlement,Greenland Landing,Andree Settlement"
+ },
+ {
+ "name": "Deuriara",
+ "pos_x": -77.875,
+ "pos_y": 105.75,
+ "pos_z": -91.21875,
+ "stations": "Laval Hub,Zhen Port,Reynolds Relay"
+ },
+ {
+ "name": "Deuringas",
+ "pos_x": 137.3125,
+ "pos_y": 3.84375,
+ "pos_z": -35.90625,
+ "stations": "Shukor Hub,Fung Orbital,Sagan Station"
+ },
+ {
+ "name": "Devadatta",
+ "pos_x": 37.3125,
+ "pos_y": -44.8125,
+ "pos_z": -133.8125,
+ "stations": "Makarov Orbital,Bruce Arsenal,Hasse Enterprise"
+ },
+ {
+ "name": "Devakak",
+ "pos_x": -67.75,
+ "pos_y": -61.5,
+ "pos_z": -14.34375,
+ "stations": "Crown Port,Meitner Platform,Dickson Mines"
+ },
+ {
+ "name": "Devaku",
+ "pos_x": 117.25,
+ "pos_y": -72.8125,
+ "pos_z": 27.34375,
+ "stations": "Howe Terminal,Dolgov Colony,Plaskett Hub,Alexander Installation"
+ },
+ {
+ "name": "Devana",
+ "pos_x": -39.5,
+ "pos_y": -97.40625,
+ "pos_z": 74.375,
+ "stations": "Greene Dock,Meinel Hub,Dittmar Hub,Ghez Orbital,Shinjo Vision,Purbach Orbital,Cowell Hub"
+ },
+ {
+ "name": "Devane",
+ "pos_x": 20.1875,
+ "pos_y": -121.1875,
+ "pos_z": -29,
+ "stations": "Spurzem Gateway,Gaughan Point,Davies City,Kimura Ring"
+ },
+ {
+ "name": "Devasu",
+ "pos_x": 8.53125,
+ "pos_y": -65.5,
+ "pos_z": -76.0625,
+ "stations": "Linnehan Landing,Kinsey Landing"
+ },
+ {
+ "name": "Devasupai",
+ "pos_x": 35.625,
+ "pos_y": -33.6875,
+ "pos_z": 115.84375,
+ "stations": "Shkaplerov Terminal,Merle Point,Kavandi Camp"
+ },
+ {
+ "name": "Devata Baru",
+ "pos_x": -133.15625,
+ "pos_y": -7.6875,
+ "pos_z": 63.5625,
+ "stations": "Saberhagen Ring,Haberlandt Plant,Evangelisti Port"
+ },
+ {
+ "name": "Devataru",
+ "pos_x": 24.28125,
+ "pos_y": 19.34375,
+ "pos_z": 90.9375,
+ "stations": "Musa al-Khwarizmi Gateway,Scott Bastion"
+ },
+ {
+ "name": "Devis",
+ "pos_x": 142.71875,
+ "pos_y": -74.09375,
+ "pos_z": 110.28125,
+ "stations": "Kulin Prospect"
+ },
+ {
+ "name": "Devithim",
+ "pos_x": 171.4375,
+ "pos_y": -66.84375,
+ "pos_z": 125.625,
+ "stations": "Muramatsu Stop,Hyecho Hub"
+ },
+ {
+ "name": "Dewi",
+ "pos_x": -40.96875,
+ "pos_y": -20.59375,
+ "pos_z": 88.5625,
+ "stations": "Zahn Landing,Longyear Estate"
+ },
+ {
+ "name": "Dewi'xil",
+ "pos_x": 57.21875,
+ "pos_y": -196.875,
+ "pos_z": 81.625,
+ "stations": "Arkhangelsky Station,Foerster Orbital,Guth Landing,Ozanne Settlement"
+ },
+ {
+ "name": "Dewi'xilaga",
+ "pos_x": 151.375,
+ "pos_y": -80.59375,
+ "pos_z": 28.1875,
+ "stations": "Quetelet Port,Naboth Settlement,Sturt Beacon"
+ },
+ {
+ "name": "Dewikum",
+ "pos_x": 19.375,
+ "pos_y": -0.28125,
+ "pos_z": -68.9375,
+ "stations": "Wyeth Platform"
+ },
+ {
+ "name": "Dewindji",
+ "pos_x": 109.25,
+ "pos_y": -1.03125,
+ "pos_z": -78.21875,
+ "stations": "Whitney Orbital,Duke Station"
+ },
+ {
+ "name": "Deyus",
+ "pos_x": -34.71875,
+ "pos_y": -51.4375,
+ "pos_z": 0.90625,
+ "stations": "Nelson Landing,Hoard Terminal,Lawson Hub"
+ },
+ {
+ "name": "Deywotho",
+ "pos_x": -140.3125,
+ "pos_y": -6.59375,
+ "pos_z": -13.875,
+ "stations": "Simpson Terminal,McDonald Beacon,Huberath Relay"
+ },
+ {
+ "name": "DH Leonis",
+ "pos_x": 29.28125,
+ "pos_y": 81.53125,
+ "pos_z": -56.34375,
+ "stations": "Boucher Market,Hugh Dock,Dyr Terminal,Brooks Base,Andrews Bastion,Eskridge Dock"
+ },
+ {
+ "name": "Dhak",
+ "pos_x": -58.09375,
+ "pos_y": -150.40625,
+ "pos_z": -7.9375,
+ "stations": "King Installation,Plait Vision,Shavyrin Vision"
+ },
+ {
+ "name": "Dhakhan",
+ "pos_x": -98.40625,
+ "pos_y": -14.46875,
+ "pos_z": -67.25,
+ "stations": "Nelson Terminal,Moskowitz Orbital"
+ },
+ {
+ "name": "Dhaku",
+ "pos_x": -71.09375,
+ "pos_y": 41.5625,
+ "pos_z": -133.875,
+ "stations": "Andrews Station,Nesvadba Holdings,Okorafor Gateway"
+ },
+ {
+ "name": "Dhakua",
+ "pos_x": 89.78125,
+ "pos_y": -182.375,
+ "pos_z": 165.84375,
+ "stations": "Iben Escape,Shirazi Escape,Hill Camp"
+ },
+ {
+ "name": "Dhakya",
+ "pos_x": -127.625,
+ "pos_y": -100.0625,
+ "pos_z": 41.53125,
+ "stations": "Virchow Port"
+ },
+ {
+ "name": "Dhan",
+ "pos_x": 0.875,
+ "pos_y": -219.46875,
+ "pos_z": 60.8125,
+ "stations": "Kidd Station,Griffiths Prospect,Osterbrock Orbital,Somayaji Prospect"
+ },
+ {
+ "name": "Dhanchu",
+ "pos_x": -118.90625,
+ "pos_y": 47.46875,
+ "pos_z": 76.34375,
+ "stations": "Schouten Port,Lerner Relay,Hyecho Colony,Cochrane Holdings"
+ },
+ {
+ "name": "Dhang",
+ "pos_x": 128.40625,
+ "pos_y": -48.5,
+ "pos_z": 60.5,
+ "stations": "Thiele City,Grunsfeld Orbital,Baille Station"
+ },
+ {
+ "name": "Dhang K'an",
+ "pos_x": 106.5,
+ "pos_y": 82.90625,
+ "pos_z": -60.5625,
+ "stations": "Forrester Hub,Gerst Landing,Rashid Works"
+ },
+ {
+ "name": "Dhang Tzela",
+ "pos_x": 110.03125,
+ "pos_y": -166.1875,
+ "pos_z": -86.875,
+ "stations": "Anderson Dock,Baker Arsenal"
+ },
+ {
+ "name": "Dhanga",
+ "pos_x": 115.96875,
+ "pos_y": -164.75,
+ "pos_z": 119.59375,
+ "stations": "Youll Legacy,Jansky Beacon"
+ },
+ {
+ "name": "Dhangba",
+ "pos_x": -97.03125,
+ "pos_y": -1.15625,
+ "pos_z": -82.1875,
+ "stations": "Francisco de Eliza Station,Veblen City,Moffitt Terminal,Blackman Reach,Coles Orbital,Gottlob Frege Point,Gibson Terminal,Poleshchuk Colony,Herbert Terminal"
+ },
+ {
+ "name": "Dhangguang",
+ "pos_x": 64.5,
+ "pos_y": -236.96875,
+ "pos_z": 46.3125,
+ "stations": "Polikarpov Station,Fehrenbach Orbital,Coulomb Arena"
+ },
+ {
+ "name": "Dhanhopi",
+ "pos_x": -6.90625,
+ "pos_y": 79.625,
+ "pos_z": -27.625,
+ "stations": "Galouye Orbital,Plucker Enterprise,Pytheas Gateway,Brothers Settlement,Witt Beacon,Rescue Ship - Plucker Enterprise"
+ },
+ {
+ "name": "Dhanka",
+ "pos_x": -70.3125,
+ "pos_y": -111.90625,
+ "pos_z": 22.53125,
+ "stations": "Bierce Horizons,Morrison Dock,Hudson Orbital,Rond d'Alembert Hub,Tavares Enterprise,Normand Vision,Powers' Inheritance,Schachner Enterprise"
+ },
+ {
+ "name": "Dhankomi",
+ "pos_x": -120,
+ "pos_y": -88.8125,
+ "pos_z": 78.8125,
+ "stations": "Rennie Beacon,Sanger Point"
+ },
+ {
+ "name": "Dhar",
+ "pos_x": 149.46875,
+ "pos_y": 76.96875,
+ "pos_z": 32.1875,
+ "stations": "Crown Stop"
+ },
+ {
+ "name": "Dharaces",
+ "pos_x": 107.6875,
+ "pos_y": 8.6875,
+ "pos_z": 120.9375,
+ "stations": "Anderson Horizons,Barnwell City,Wisniewski-Snerg Vision,Fairbairn Depot"
+ },
+ {
+ "name": "Dharai",
+ "pos_x": -106.875,
+ "pos_y": 27.65625,
+ "pos_z": 32.65625,
+ "stations": "Littlewood Terminal,Williams City,Lebesgue Enterprise"
+ },
+ {
+ "name": "Dharani",
+ "pos_x": 138.53125,
+ "pos_y": -66.9375,
+ "pos_z": -74.375,
+ "stations": "Merritt Mines"
+ },
+ {
+ "name": "Dharasir",
+ "pos_x": 21.15625,
+ "pos_y": -100.90625,
+ "pos_z": 18.09375,
+ "stations": "Hickam Terminal,Bonestell Dock"
+ },
+ {
+ "name": "Dharawal",
+ "pos_x": -106.125,
+ "pos_y": 2.65625,
+ "pos_z": 73.09375,
+ "stations": "Rusch Works"
+ },
+ {
+ "name": "Dharelbici",
+ "pos_x": -144.28125,
+ "pos_y": -14.1875,
+ "pos_z": 49.53125,
+ "stations": "Verne Mines,Davis Platform"
+ },
+ {
+ "name": "Dharina",
+ "pos_x": -122.375,
+ "pos_y": -21.5625,
+ "pos_z": 39.6875,
+ "stations": "Manakov Orbital,Scheutz Enterprise,Haise Dock,Shepherd Holdings,Hahn Landing"
+ },
+ {
+ "name": "Dharipai",
+ "pos_x": -95.03125,
+ "pos_y": 44.53125,
+ "pos_z": 32.4375,
+ "stations": "Ray Settlement,Kendrick Plant,Henderson Asylum"
+ },
+ {
+ "name": "Dharm",
+ "pos_x": -60.40625,
+ "pos_y": 15.78125,
+ "pos_z": 78.65625,
+ "stations": "Sacco Dock"
+ },
+ {
+ "name": "Dharragense",
+ "pos_x": -46.03125,
+ "pos_y": 58.0625,
+ "pos_z": -93.5,
+ "stations": "Tiedemann Horizons,Lewitt Installation,Vardeman Survey"
+ },
+ {
+ "name": "Dharuek",
+ "pos_x": -43.125,
+ "pos_y": -14.40625,
+ "pos_z": 104.9375,
+ "stations": "Tarski Arsenal,Dummer Dock,Bykovsky Works,Vaugh Depot,Cartan Vision"
+ },
+ {
+ "name": "Dharuwar",
+ "pos_x": 142.90625,
+ "pos_y": 74.375,
+ "pos_z": 35.90625,
+ "stations": "Lehtonen Holdings,Slusser Orbital,Fourier Silo,Kube-McDowell Beacon"
+ },
+ {
+ "name": "Dhata",
+ "pos_x": -22.6875,
+ "pos_y": 133.90625,
+ "pos_z": -30.40625,
+ "stations": "Cleve Terminal,Forsskal Laboratory,Houtman Dock,Hambly Keep"
+ },
+ {
+ "name": "Dhathaarib",
+ "pos_x": 68.53125,
+ "pos_y": 28.21875,
+ "pos_z": 45.46875,
+ "stations": "Berezovoy Terminal,Mills Settlement"
+ },
+ {
+ "name": "Dhathaut",
+ "pos_x": -23.625,
+ "pos_y": 61,
+ "pos_z": 106.46875,
+ "stations": "Helms Hub,Goddard Port,Viehbock Hub,Macarthur Gateway,Lu Station,Crown Dock,Weiss Arsenal,Blaha Holdings,Serebrov Vision,Busch's Pride"
+ },
+ {
+ "name": "Dheneb",
+ "pos_x": -24.9375,
+ "pos_y": -118,
+ "pos_z": -28.625,
+ "stations": "Henson Gateway,Paczynski Beacon,Viete Point"
+ },
+ {
+ "name": "Dhodia",
+ "pos_x": 6.65625,
+ "pos_y": 25.625,
+ "pos_z": 107.21875,
+ "stations": "Crown Survey,Fourier Enterprise,Poncelet Dock,Loncke Base,Hodgson City"
+ },
+ {
+ "name": "Dhor",
+ "pos_x": -25.53125,
+ "pos_y": 35,
+ "pos_z": 154.375,
+ "stations": "Lagrange Landing,Litke Prospect"
+ },
+ {
+ "name": "Dhorai",
+ "pos_x": 91.46875,
+ "pos_y": 54.71875,
+ "pos_z": -11.75,
+ "stations": "Navigator Installation"
+ },
+ {
+ "name": "Dhrites",
+ "pos_x": 131.75,
+ "pos_y": -84.125,
+ "pos_z": -50.125,
+ "stations": "Kresak Reach,Lintott Stop"
+ },
+ {
+ "name": "Dhrutin",
+ "pos_x": -62.5625,
+ "pos_y": -42.4375,
+ "pos_z": 5.125,
+ "stations": "Chorel Terminal"
+ },
+ {
+ "name": "Dhruva",
+ "pos_x": 76.28125,
+ "pos_y": -134.75,
+ "pos_z": 118.75,
+ "stations": "Shinjo City,Moon Penal colony"
+ },
+ {
+ "name": "Di Jian",
+ "pos_x": -16.5625,
+ "pos_y": 108.25,
+ "pos_z": 5.34375,
+ "stations": "Preuss Terminal,Rescue Ship - Preuss Terminal"
+ },
+ {
+ "name": "Di Kian",
+ "pos_x": 33.0625,
+ "pos_y": 158.375,
+ "pos_z": -59.84375,
+ "stations": "Mendez Survey"
+ },
+ {
+ "name": "Di Kuana",
+ "pos_x": -35.75,
+ "pos_y": -37.78125,
+ "pos_z": 100.59375,
+ "stations": "Macgregor City,Tilley Enterprise,Fearn Ring,Dyr Terminal,Citroen Vision,Gunn Terminal,Ostrander City,Flynn Legacy"
+ },
+ {
+ "name": "Di Kun",
+ "pos_x": 128.625,
+ "pos_y": -88.46875,
+ "pos_z": 6.25,
+ "stations": "Bigourdan Platform,Porco Landing"
+ },
+ {
+ "name": "Di Yi",
+ "pos_x": -45.90625,
+ "pos_y": -61.78125,
+ "pos_z": -116.375,
+ "stations": "Fraser Settlement,Forbes Dock"
+ },
+ {
+ "name": "Di Yomi",
+ "pos_x": 104.59375,
+ "pos_y": -178.84375,
+ "pos_z": 57.75,
+ "stations": "Kelly Orbital,Maanen Station,Artzybasheff Terminal,Graham Settlement,Shimizu Terminal,Emshwiller Orbital,Mason Hub,Vaisala Orbital"
+ },
+ {
+ "name": "Di Yomis",
+ "pos_x": 184.21875,
+ "pos_y": -120.875,
+ "pos_z": 88.15625,
+ "stations": "Chernykh Hub,Rich Dock,Linge Survey"
+ },
+ {
+ "name": "Diaba",
+ "pos_x": 164,
+ "pos_y": -99.6875,
+ "pos_z": 6.96875,
+ "stations": "Niijima Station,Anthony de la Roche Horizons,Pryor Keep"
+ },
+ {
+ "name": "Diabak",
+ "pos_x": 35.84375,
+ "pos_y": -22,
+ "pos_z": -24.15625,
+ "stations": "Bean Beacon,Pryor Enterprise,Daimler Ring,Chretien Dock,Hartog Horizons,Thomson Orbital,Rennie Ring,Furrer Enterprise,Stefanyshyn-Piper Port,Bridges Survey"
+ },
+ {
+ "name": "Diabanda",
+ "pos_x": 91.21875,
+ "pos_y": -25.96875,
+ "pos_z": -57.53125,
+ "stations": "Kapp Dock,Everest Co-operative,Kondakova Penal colony,Muhammad Ibn Battuta Port"
+ },
+ {
+ "name": "Diabingo",
+ "pos_x": 15.3125,
+ "pos_y": -142.875,
+ "pos_z": 152.125,
+ "stations": "Al Sufi Settlement"
+ },
+ {
+ "name": "Diabozo",
+ "pos_x": -159.34375,
+ "pos_y": 28.9375,
+ "pos_z": -89.03125,
+ "stations": "Abbott Plant,Pudwill Gorie Settlement,Zhen Terminal"
+ },
+ {
+ "name": "Diaguan",
+ "pos_x": -122.9375,
+ "pos_y": -24.46875,
+ "pos_z": -60.78125,
+ "stations": "Jemison Mines"
+ },
+ {
+ "name": "Diaguandri",
+ "pos_x": -41.0625,
+ "pos_y": -62.15625,
+ "pos_z": -103.25,
+ "stations": "Ray Gateway,Rothman Vista"
+ },
+ {
+ "name": "Diaguthivar",
+ "pos_x": -8.6875,
+ "pos_y": 2.0625,
+ "pos_z": -108.375,
+ "stations": "Qurra Landing,Napier Plant,Offutt Enterprise"
+ },
+ {
+ "name": "Diakas",
+ "pos_x": -20.96875,
+ "pos_y": 76,
+ "pos_z": -97.625,
+ "stations": "Huberath Terminal"
+ },
+ {
+ "name": "Dian",
+ "pos_x": 48.375,
+ "pos_y": -105.34375,
+ "pos_z": -80.65625,
+ "stations": "Lilienthal Orbital,Fallows Orbital,Calatrava Orbital"
+ },
+ {
+ "name": "Dian Cecht",
+ "pos_x": -9.3125,
+ "pos_y": 1.03125,
+ "pos_z": 132.46875,
+ "stations": "Lichtenberg Plant,Drexler Colony"
+ },
+ {
+ "name": "Didarengu",
+ "pos_x": 27.375,
+ "pos_y": 18.1875,
+ "pos_z": -89.46875,
+ "stations": "Watt Settlement,Bolotov Gateway,Hutton Dock,Payson Base"
+ },
+ {
+ "name": "Didavas",
+ "pos_x": 99.71875,
+ "pos_y": -10.78125,
+ "pos_z": 42.25,
+ "stations": "Culpeper Landing,Henslow Arsenal,Hahn Plant,Zettel's Progress"
+ },
+ {
+ "name": "Didawar",
+ "pos_x": 113.375,
+ "pos_y": -118.3125,
+ "pos_z": 145.3125,
+ "stations": "Babcock Dock,Filippenko Stop,Goryu Prospect"
+ },
+ {
+ "name": "Didayac",
+ "pos_x": 27.3125,
+ "pos_y": -42.875,
+ "pos_z": -44.84375,
+ "stations": "Smith Beacon"
+ },
+ {
+ "name": "Didio",
+ "pos_x": 73,
+ "pos_y": 68,
+ "pos_z": 33.03125,
+ "stations": "Weyn Orbital,Laumer Orbital,Greenleaf Gateway,Steele Dock,Comer Hub,Burnham Gateway,Caryanda Dock,Cousteau Survey,Walker Observatory"
+ },
+ {
+ "name": "Didiomanja",
+ "pos_x": 77.6875,
+ "pos_y": -73.8125,
+ "pos_z": -91.09375,
+ "stations": "Babakin Orbital,Kreutz Hub,Leydenfrost Dock,Alcock City,Herndon Point,Marcy Terminal,Herjulfsson Exchange"
+ },
+ {
+ "name": "Diegakul",
+ "pos_x": 88.59375,
+ "pos_y": -24.03125,
+ "pos_z": 84.75,
+ "stations": "Diesel Orbital,Wallace Orbital,Dampier Survey"
+ },
+ {
+ "name": "Dierfar",
+ "pos_x": 73.375,
+ "pos_y": 38.71875,
+ "pos_z": 10.8125,
+ "stations": "Joule Orbital,Ciferri Dock,Lazarev Hub,Galvani Port,Baker Station,Bean Dock,Cavendish Installation,Evans Port,Clifford Holdings,Linteris Gateway,Thompson Market"
+ },
+ {
+ "name": "Dierites",
+ "pos_x": 23.6875,
+ "pos_y": -75.96875,
+ "pos_z": -17.03125,
+ "stations": "Leoniceno City,Volynov Orbital,Zhen City,Cixin Gateway,Wetherbee Port"
+ },
+ {
+ "name": "Dieslic",
+ "pos_x": -112.375,
+ "pos_y": 0.21875,
+ "pos_z": -58.25,
+ "stations": "Fernandez Works,Verrazzano Hub,Benford Landing"
+ },
+ {
+ "name": "Dietri",
+ "pos_x": -5.375,
+ "pos_y": 71.5625,
+ "pos_z": -54.25,
+ "stations": "Wilcutt Station,Evans Market"
+ },
+ {
+ "name": "Dievantja",
+ "pos_x": 160.9375,
+ "pos_y": -119.375,
+ "pos_z": 79.84375,
+ "stations": "Husband Mine"
+ },
+ {
+ "name": "Dievat",
+ "pos_x": 85.53125,
+ "pos_y": -84.375,
+ "pos_z": -3.125,
+ "stations": "Langley Gateway,Bothezat City"
+ },
+ {
+ "name": "Difu",
+ "pos_x": -34.40625,
+ "pos_y": 1.4375,
+ "pos_z": -27.875,
+ "stations": "Backers Pledge High,Frobac's Hope,Oswald Colony,Bunsen Orbital,McArthur Horizons"
+ },
+ {
+ "name": "Diggidiggi",
+ "pos_x": -9518.34375,
+ "pos_y": -893.09375,
+ "pos_z": 19822.90625,
+ "stations": "Bascom's Pride,Stein Works"
+ },
+ {
+ "name": "Dijkstra",
+ "pos_x": 103.75,
+ "pos_y": 57.46875,
+ "pos_z": 73.375,
+ "stations": "Allen Platform,Lienward,Bolger Holdings"
+ },
+ {
+ "name": "Dijuhte",
+ "pos_x": 65.0625,
+ "pos_y": -251.21875,
+ "pos_z": -25.59375,
+ "stations": "Kreutz Terminal,Pickering Terminal,Humphreys Beacon"
+ },
+ {
+ "name": "Dilati",
+ "pos_x": -15.1875,
+ "pos_y": 64.71875,
+ "pos_z": -64.09375,
+ "stations": "Dezhurov Terminal,Reamy Settlement"
+ },
+ {
+ "name": "Dilga",
+ "pos_x": 10.125,
+ "pos_y": -124.9375,
+ "pos_z": 25.6875,
+ "stations": "Flettner Depot,Ghez Dock,Perrine Orbital"
+ },
+ {
+ "name": "Dilgaenses",
+ "pos_x": 135.6875,
+ "pos_y": -220.09375,
+ "pos_z": 15.9375,
+ "stations": "MacCready Reach,Andoyer Bastion"
+ },
+ {
+ "name": "Dilgariang",
+ "pos_x": 32.84375,
+ "pos_y": -47.5625,
+ "pos_z": -72.90625,
+ "stations": "Still Installation"
+ },
+ {
+ "name": "Dilwa",
+ "pos_x": -133.90625,
+ "pos_y": -1.21875,
+ "pos_z": -33.375,
+ "stations": "Scott Relay,Malocello's Claim"
+ },
+ {
+ "name": "Dilwanes",
+ "pos_x": 120.4375,
+ "pos_y": 16.125,
+ "pos_z": -87.84375,
+ "stations": "Clauss Dock,Jenkinson Dock,Powell Mines,Quick Terminal,Kirk Prospect,Eckford Beacon"
+ },
+ {
+ "name": "Dilwicani",
+ "pos_x": 93.4375,
+ "pos_y": -189.28125,
+ "pos_z": 5.25,
+ "stations": "Woodroffe's Claim,Kondo Silo"
+ },
+ {
+ "name": "Dimai",
+ "pos_x": 91.21875,
+ "pos_y": 34.625,
+ "pos_z": -106.84375,
+ "stations": "Ryumin Station,Coulomb Plant,Volk Mines"
+ },
+ {
+ "name": "Dimaratem",
+ "pos_x": -184.375,
+ "pos_y": 70.9375,
+ "pos_z": 9.84375,
+ "stations": "Kennicott Vision,Rustah Landing,Robinson Relay"
+ },
+ {
+ "name": "Dimoco",
+ "pos_x": -73.625,
+ "pos_y": 22.15625,
+ "pos_z": 59.1875,
+ "stations": "Meitner Ring,Edison Orbital"
+ },
+ {
+ "name": "Dimocorna",
+ "pos_x": 20.3125,
+ "pos_y": -51.4375,
+ "pos_z": 165.375,
+ "stations": "Burton Vision,Geston Bastion,Popov Vision"
+ },
+ {
+ "name": "Dimor",
+ "pos_x": 111.25,
+ "pos_y": -80.5625,
+ "pos_z": 25.71875,
+ "stations": "Aoki Terminal"
+ },
+ {
+ "name": "Dimshi",
+ "pos_x": -21.15625,
+ "pos_y": -163.34375,
+ "pos_z": 18.40625,
+ "stations": "Naubakht Prospect,Barr Reach,von Bellingshausen Depot"
+ },
+ {
+ "name": "Dimste",
+ "pos_x": 70.71875,
+ "pos_y": -216.84375,
+ "pos_z": -16.8125,
+ "stations": "Hussenot Reach"
+ },
+ {
+ "name": "Dimsti",
+ "pos_x": 63.125,
+ "pos_y": -72.59375,
+ "pos_z": 117.15625,
+ "stations": "Bliss Colony,Henderson Terminal,Wendelin Landing"
+ },
+ {
+ "name": "Dinda",
+ "pos_x": -21.9375,
+ "pos_y": -52.15625,
+ "pos_z": -66.625,
+ "stations": "Jensen Keep,Yeliseyev Station,Mount Sunday,Wakata Keep,Ravenclan's Astro Lab"
+ },
+ {
+ "name": "Dindbhai",
+ "pos_x": -134.9375,
+ "pos_y": -55.0625,
+ "pos_z": 81.3125,
+ "stations": "MacLean Port,Bean Beacon"
+ },
+ {
+ "name": "Dinde",
+ "pos_x": 73.625,
+ "pos_y": -229.40625,
+ "pos_z": -24.71875,
+ "stations": "Wallis Outpost,Youll Penal colony"
+ },
+ {
+ "name": "Dindjeris",
+ "pos_x": 123.28125,
+ "pos_y": 66.25,
+ "pos_z": 29.78125,
+ "stations": "Fossum Horizons,Chamitoff Vision,Menzies Settlement,Ferguson Landing,Mieville Keep"
+ },
+ {
+ "name": "Dinkas",
+ "pos_x": 19.40625,
+ "pos_y": -163.1875,
+ "pos_z": 77.5625,
+ "stations": "Asami Station,Blaauw Port,Cantor Prospect,Young Enterprise"
+ },
+ {
+ "name": "Diomawi",
+ "pos_x": -46.4375,
+ "pos_y": -127.875,
+ "pos_z": 111.28125,
+ "stations": "de Andrade Vision,Lopez Vision,Gould Prospect,Kanai Hub"
+ },
+ {
+ "name": "Diousteri",
+ "pos_x": 80.9375,
+ "pos_y": 107.15625,
+ "pos_z": -35.125,
+ "stations": "Born Dock,Cayley Terminal,Cugnot Hangar,Kovalyonok Orbital"
+ },
+ {
+ "name": "Dirone",
+ "pos_x": 77.25,
+ "pos_y": -44.75,
+ "pos_z": -99.375,
+ "stations": "Humphreys Dock,Zetford Platform,Leonov Prospect,Fettman Base"
+ },
+ {
+ "name": "Dironii",
+ "pos_x": -33,
+ "pos_y": -171.40625,
+ "pos_z": 59.3125,
+ "stations": "Gentil Station,Mayer Enterprise,MacDonald Prospect"
+ },
+ {
+ "name": "Dis Pater",
+ "pos_x": -3.15625,
+ "pos_y": -66.40625,
+ "pos_z": 95.6875,
+ "stations": "Lazutkin Gateway,Wallace Terminal,Drzewiecki's Claim"
+ },
+ {
+ "name": "Disci",
+ "pos_x": 16.03125,
+ "pos_y": 97.59375,
+ "pos_z": -29.59375,
+ "stations": "Snodgrass Orbital"
+ },
+ {
+ "name": "Diso",
+ "pos_x": 72.15625,
+ "pos_y": 48.75,
+ "pos_z": 70.75,
+ "stations": "Bao Station,Baker Hub,Shifnalport,Raleigh Legacy,Gloss Lab"
+ },
+ {
+ "name": "Ditae",
+ "pos_x": 0.9375,
+ "pos_y": -157.34375,
+ "pos_z": -6.21875,
+ "stations": "Maupertuis Depot,Langley Dock,Mattingly Vision,Matthews Horizons"
+ },
+ {
+ "name": "Ditia",
+ "pos_x": 32.46875,
+ "pos_y": -39.59375,
+ "pos_z": -36.5,
+ "stations": "Zetford Base,Arkwright City,Bosch Point"
+ },
+ {
+ "name": "Ditian",
+ "pos_x": 97.4375,
+ "pos_y": -89.09375,
+ "pos_z": 65.96875,
+ "stations": "Fan Gateway,Banneker Installation,Mukai Orbital,Sugano Terminal"
+ },
+ {
+ "name": "Ditibi",
+ "pos_x": 28.9375,
+ "pos_y": -11.53125,
+ "pos_z": 3.46875,
+ "stations": "Serebrov Gateway,Zhen Gateway,Conrad Dock,Jones Gateway,Priestley Enterprise,Babbage City,Drexler Installation,Volk Enterprise,Crown Refinery,Carrier Ring,Biggle Observatory,Nespoli Orbital"
+ },
+ {
+ "name": "Diticush",
+ "pos_x": 91.6875,
+ "pos_y": -101.15625,
+ "pos_z": -86.84375,
+ "stations": "Lobachevsky Station,Slade Ring,Malocello Colony"
+ },
+ {
+ "name": "Diviriks",
+ "pos_x": 129.125,
+ "pos_y": 4.4375,
+ "pos_z": 105.21875,
+ "stations": "McMonagle Ring,Seddon City,Wilson Survey"
+ },
+ {
+ "name": "Divja Mocha",
+ "pos_x": 58.625,
+ "pos_y": -207.9375,
+ "pos_z": 49.15625,
+ "stations": "Sweet Orbital,Piccard Bastion,Brashear Vision,Nicholson Gateway"
+ },
+ {
+ "name": "Divja Mu",
+ "pos_x": -92.3125,
+ "pos_y": 13.4375,
+ "pos_z": -16.8125,
+ "stations": "Coles Mines,Knight Landing,Zetford's Inheritance"
+ },
+ {
+ "name": "Djabal",
+ "pos_x": 150.84375,
+ "pos_y": 0.71875,
+ "pos_z": 21.875,
+ "stations": "Macarthur's Inheritance,Parmitano Mine"
+ },
+ {
+ "name": "Djabara",
+ "pos_x": 90.28125,
+ "pos_y": -237.4375,
+ "pos_z": 57.25,
+ "stations": "Bauman Relay,Potez Beacon,Mitchell Relay"
+ },
+ {
+ "name": "Djabe",
+ "pos_x": 35.25,
+ "pos_y": -88.25,
+ "pos_z": 76.40625,
+ "stations": "Schumacher Estate,d'Allonville Vision"
+ },
+ {
+ "name": "Djabea",
+ "pos_x": 123.78125,
+ "pos_y": -144.8125,
+ "pos_z": 125.25,
+ "stations": "Meuron Vision,Ikeya Hub,Bolton Orbital,Crown Camp"
+ },
+ {
+ "name": "Djabelmalo",
+ "pos_x": -88.25,
+ "pos_y": -86.03125,
+ "pos_z": -115.75,
+ "stations": "Barbosa's Progress,Carey Camp,Gillekens Beacon"
+ },
+ {
+ "name": "Djabijabus",
+ "pos_x": -57.34375,
+ "pos_y": -99.59375,
+ "pos_z": 118.53125,
+ "stations": "Collins Holdings,Veach Enterprise"
+ },
+ {
+ "name": "Djabugandji",
+ "pos_x": 1.71875,
+ "pos_y": 34.78125,
+ "pos_z": 119.34375,
+ "stations": "Deb Station"
+ },
+ {
+ "name": "Djabushis",
+ "pos_x": -30.96875,
+ "pos_y": -31.90625,
+ "pos_z": 34.0625,
+ "stations": "Cheli Terminal"
+ },
+ {
+ "name": "Djagana",
+ "pos_x": 43.84375,
+ "pos_y": -44.625,
+ "pos_z": -149.25,
+ "stations": "Bertin Settlement,Oxley Terminal"
+ },
+ {
+ "name": "Djagh",
+ "pos_x": 84.125,
+ "pos_y": -215.96875,
+ "pos_z": -3.625,
+ "stations": "Lee Camp"
+ },
+ {
+ "name": "Djaghire",
+ "pos_x": 141.5,
+ "pos_y": -173.75,
+ "pos_z": 23.34375,
+ "stations": "Nojiri Port,Nourse Survey,Bell Orbital,Poyser Hub,Sato Vision,Robert Terminal,Safdie Station,Brom Hub,Piazzi Port,Eisinga Hub,Somayaji Mines,Kratman Vision,O'Donnell Lab"
+ },
+ {
+ "name": "Djagho",
+ "pos_x": -58.8125,
+ "pos_y": -161.75,
+ "pos_z": -20.375,
+ "stations": "Ortiz Moreno Oasis"
+ },
+ {
+ "name": "Djaghom Dun",
+ "pos_x": -32.34375,
+ "pos_y": 155.21875,
+ "pos_z": -31.8125,
+ "stations": "Yolen Installation,Toll Landing"
+ },
+ {
+ "name": "Djak",
+ "pos_x": 27.65625,
+ "pos_y": 26.8125,
+ "pos_z": 74.6875,
+ "stations": "Fischer's Claim,McMonagle's Claim,Leestma Terminal"
+ },
+ {
+ "name": "Djakah",
+ "pos_x": 105.53125,
+ "pos_y": -134.46875,
+ "pos_z": 101.25,
+ "stations": "Al-Khujandi Hub,Schwarzschild Vision,Youll Survey,Sandage Vista,Bottego Reach"
+ },
+ {
+ "name": "Djakam",
+ "pos_x": 24.1875,
+ "pos_y": 80.5,
+ "pos_z": -60.09375,
+ "stations": "Porsche Hub"
+ },
+ {
+ "name": "Djakunda",
+ "pos_x": -40.4375,
+ "pos_y": -90.4375,
+ "pos_z": -34.375,
+ "stations": "Cernan Settlement,Hedley Camp"
+ },
+ {
+ "name": "Djalakuru",
+ "pos_x": -70.5625,
+ "pos_y": -98.21875,
+ "pos_z": 124.625,
+ "stations": "Vancouver Orbital,Wilder Enterprise,Baker Holdings,Baker City,Houtman Settlement,Moriarty's Inheritance"
+ },
+ {
+ "name": "Djalibara",
+ "pos_x": 2.40625,
+ "pos_y": -97.46875,
+ "pos_z": 46,
+ "stations": "Mozhaysky Gateway,Mattingly Vision"
+ },
+ {
+ "name": "Djalkyries",
+ "pos_x": 110.3125,
+ "pos_y": -154,
+ "pos_z": 100.875,
+ "stations": "Boscovich Survey,Phillifent's Pride,Tusi Dock,Millosevich Hub,Zaschka Landing"
+ },
+ {
+ "name": "Djamaygani",
+ "pos_x": 65.78125,
+ "pos_y": 95.65625,
+ "pos_z": 82.0625,
+ "stations": "Kirtley Ring,Hardy Port,Siegel's Progress,Cleve Dock,Larbalestier Depot"
+ },
+ {
+ "name": "Djambarex",
+ "pos_x": -1.375,
+ "pos_y": -17.375,
+ "pos_z": 140.65625,
+ "stations": "Coppel Silo,Davidson Orbital,Cleve Beacon"
+ },
+ {
+ "name": "Djambe",
+ "pos_x": -56.5625,
+ "pos_y": 4.53125,
+ "pos_z": 156.90625,
+ "stations": "Brust Port,Wallin Station,Plexico Colony"
+ },
+ {
+ "name": "Djambiradh",
+ "pos_x": 28.53125,
+ "pos_y": -89.09375,
+ "pos_z": 37.4375,
+ "stations": "Tago Stop"
+ },
+ {
+ "name": "Djambojai",
+ "pos_x": 64.25,
+ "pos_y": 49.125,
+ "pos_z": -142.5625,
+ "stations": "Shuttleworth Point,Lazarev Base,Russell Base"
+ },
+ {
+ "name": "Djamburii",
+ "pos_x": 14.8125,
+ "pos_y": -181.40625,
+ "pos_z": 50.46875,
+ "stations": "Schaumasse Port,McManus Landing,Springer Base"
+ },
+ {
+ "name": "Djamitra",
+ "pos_x": 33.6875,
+ "pos_y": 11.5625,
+ "pos_z": 99.9375,
+ "stations": "Bohm Plant,Lazarev Survey"
+ },
+ {
+ "name": "Djand",
+ "pos_x": 3.25,
+ "pos_y": -44.0625,
+ "pos_z": -92.1875,
+ "stations": "Perry Station,Burnet Lab,Hamilton Survey"
+ },
+ {
+ "name": "Djandandrun",
+ "pos_x": -104.53125,
+ "pos_y": -56.0625,
+ "pos_z": -55.75,
+ "stations": "Tanaka Dock,Dashiell Refinery"
+ },
+ {
+ "name": "Djandji",
+ "pos_x": 18.96875,
+ "pos_y": -54.90625,
+ "pos_z": -11.84375,
+ "stations": "Ellis Dock,Watson Platform,Mastracchio Keep"
+ },
+ {
+ "name": "Djangandra",
+ "pos_x": -21.40625,
+ "pos_y": -219.9375,
+ "pos_z": 89.21875,
+ "stations": "Payne-Scott Works"
+ },
+ {
+ "name": "Djanharu",
+ "pos_x": 136.46875,
+ "pos_y": -20.0625,
+ "pos_z": -40.625,
+ "stations": "Midgeley Depot,Fujimori Landing,Sharman Platform"
+ },
+ {
+ "name": "Djapan",
+ "pos_x": -171.0625,
+ "pos_y": -20.71875,
+ "pos_z": -5.78125,
+ "stations": "Al-Kashi Legacy"
+ },
+ {
+ "name": "Djapati",
+ "pos_x": 60.875,
+ "pos_y": -34.09375,
+ "pos_z": 27.25,
+ "stations": "Linteris Stop,Sinclair Base"
+ },
+ {
+ "name": "Djara",
+ "pos_x": 150.125,
+ "pos_y": -19.90625,
+ "pos_z": -100.75,
+ "stations": "Laplace Port,Fullerton Relay"
+ },
+ {
+ "name": "Djaracates",
+ "pos_x": 56.3125,
+ "pos_y": -189.34375,
+ "pos_z": 89.4375,
+ "stations": "Tisserand Sanctuary,Regiomontanus Port"
+ },
+ {
+ "name": "Djari",
+ "pos_x": -54.3125,
+ "pos_y": -76.84375,
+ "pos_z": 151.25,
+ "stations": "Tapinas Orbital"
+ },
+ {
+ "name": "Djau",
+ "pos_x": 7.59375,
+ "pos_y": -32.5625,
+ "pos_z": 86.90625,
+ "stations": "Oluwafemi Terminal,Popovich Barracks,Freud Port,Arrhenius Dock,Hopkins Town"
+ },
+ {
+ "name": "Djaui",
+ "pos_x": -80.53125,
+ "pos_y": -17.6875,
+ "pos_z": 107.0625,
+ "stations": "Krupkat Outpost,Mieville Terminal"
+ },
+ {
+ "name": "Djaujang",
+ "pos_x": -11.1875,
+ "pos_y": -70.5625,
+ "pos_z": -98.4375,
+ "stations": "Cochrane Refinery,Martins Prospect"
+ },
+ {
+ "name": "Djaujas",
+ "pos_x": -69.09375,
+ "pos_y": 75.6875,
+ "pos_z": 10.21875,
+ "stations": "Cogswell Observatory,Daley City,von Krusenstern Enterprise,Janszoon Arsenal"
+ },
+ {
+ "name": "Djedet",
+ "pos_x": -92.84375,
+ "pos_y": 22.8125,
+ "pos_z": -22.3125,
+ "stations": "Thoreau Platform,Linnaeus Hangar,Barbaro Installation"
+ },
+ {
+ "name": "Djen",
+ "pos_x": 113.4375,
+ "pos_y": -170.5625,
+ "pos_z": -5.09375,
+ "stations": "Gonnessiat Prospect"
+ },
+ {
+ "name": "Djendege",
+ "pos_x": 110.3125,
+ "pos_y": -175.65625,
+ "pos_z": -52.96875,
+ "stations": "Xuesen Relay"
+ },
+ {
+ "name": "Djendelis",
+ "pos_x": 12.21875,
+ "pos_y": 11.59375,
+ "pos_z": 69.6875,
+ "stations": "Atwater Enterprise,Simonyi City,Faris Ring,Becquerel Legacy,Sacco Beacon,Ings' Progress"
+ },
+ {
+ "name": "Djenn Crua",
+ "pos_x": 57.125,
+ "pos_y": -82.21875,
+ "pos_z": -49.125,
+ "stations": "Mil Terminal,Schommer Refinery,Sweet Horizons,Smith Escape"
+ },
+ {
+ "name": "Djenne",
+ "pos_x": 15.375,
+ "pos_y": -35.5,
+ "pos_z": -88.875,
+ "stations": "Bobko Depot"
+ },
+ {
+ "name": "Djenni",
+ "pos_x": 126.15625,
+ "pos_y": -66.6875,
+ "pos_z": -3.1875,
+ "stations": "Woolley Port,Thome Installation,Sudworth Terminal"
+ },
+ {
+ "name": "Djera",
+ "pos_x": 123.875,
+ "pos_y": -177.875,
+ "pos_z": -9.65625,
+ "stations": "Scotti Dock,Rizvi's Folly,Chertovsky Dock,Nadiradze Survey,McNaught Refinery,Mustelin Works"
+ },
+ {
+ "name": "Djerait",
+ "pos_x": 163.1875,
+ "pos_y": -117.90625,
+ "pos_z": -1.78125,
+ "stations": "Nakano Mines,Trujillo Prospect"
+ },
+ {
+ "name": "Djeranses",
+ "pos_x": 96.34375,
+ "pos_y": -63.03125,
+ "pos_z": -40.03125,
+ "stations": "Rorschach Dock,Hauck Gateway,Murray Terminal"
+ },
+ {
+ "name": "Djerato",
+ "pos_x": 151.90625,
+ "pos_y": -216.28125,
+ "pos_z": 31.5,
+ "stations": "McIntosh Horizons,Zubrin Holdings,Shinjo Landing,Champlain Relay"
+ },
+ {
+ "name": "Djeriman",
+ "pos_x": 137.4375,
+ "pos_y": 26.125,
+ "pos_z": -45.78125,
+ "stations": "Ivins Port,Stebler City,Wankel Hub"
+ },
+ {
+ "name": "Djilak",
+ "pos_x": 111.3125,
+ "pos_y": -171.71875,
+ "pos_z": -0.4375,
+ "stations": "Marriott Port,Flaugergues Mine"
+ },
+ {
+ "name": "Djinajeri",
+ "pos_x": 103.375,
+ "pos_y": -1.96875,
+ "pos_z": 112.90625,
+ "stations": "Simpson Orbital,Binder Settlement"
+ },
+ {
+ "name": "Djinaura",
+ "pos_x": -91.03125,
+ "pos_y": -36.75,
+ "pos_z": -60.5625,
+ "stations": "Murphy Terminal,Willis Park,Chalker Relay"
+ },
+ {
+ "name": "Djinba",
+ "pos_x": -118.375,
+ "pos_y": -48.28125,
+ "pos_z": -63,
+ "stations": "al-Khowarizmi Station,Grant Terminal,Tiedemann City,Person Dock,Walker Orbital,Valdes Barracks,Taylor Refinery"
+ },
+ {
+ "name": "Djinbin",
+ "pos_x": 128.96875,
+ "pos_y": -20.25,
+ "pos_z": -64.34375,
+ "stations": "Galiano Plant,Mills Installation,Cummings Works"
+ },
+ {
+ "name": "Djira",
+ "pos_x": 181.8125,
+ "pos_y": -132.78125,
+ "pos_z": 31.78125,
+ "stations": "Xuesen Ring,Bond Station,Mallett Terminal,van de Hulst Hub"
+ },
+ {
+ "name": "Djirahgwe",
+ "pos_x": -130.46875,
+ "pos_y": 38.6875,
+ "pos_z": 80.03125,
+ "stations": "Binnie Survey"
+ },
+ {
+ "name": "Djiraja",
+ "pos_x": 172.625,
+ "pos_y": -54.46875,
+ "pos_z": 65.90625,
+ "stations": "Gentil Port,Herschel Hub,Bellamy's Progress"
+ },
+ {
+ "name": "Djiratians",
+ "pos_x": 124.9375,
+ "pos_y": -138.5,
+ "pos_z": 64.09375,
+ "stations": "Chacornac Beacon"
+ },
+ {
+ "name": "Djirbalngan",
+ "pos_x": -62.75,
+ "pos_y": -61,
+ "pos_z": 111.4375,
+ "stations": "Nicollet Dock,Galiano Enterprise"
+ },
+ {
+ "name": "Djiruach",
+ "pos_x": 130.875,
+ "pos_y": -106.625,
+ "pos_z": -73.5625,
+ "stations": "Siegel Station,Morrow Ring,Verrier Terminal,Poisson Survey"
+ },
+ {
+ "name": "Djirupatha",
+ "pos_x": -7.28125,
+ "pos_y": 70.53125,
+ "pos_z": -135.25,
+ "stations": "Auld Platform"
+ },
+ {
+ "name": "Djiwa",
+ "pos_x": 80.84375,
+ "pos_y": -147.03125,
+ "pos_z": 137.125,
+ "stations": "Juan de la Cierva Terminal,Gabrielli Dock"
+ },
+ {
+ "name": "Djiwal",
+ "pos_x": 119.53125,
+ "pos_y": -0.125,
+ "pos_z": -41.0625,
+ "stations": "Thompson Dock,White Station,Bounds Gateway,Payne City,Barbosa Orbital,Amnuel Orbital,Galouye Dock"
+ },
+ {
+ "name": "Djiwali",
+ "pos_x": 58.15625,
+ "pos_y": 37.6875,
+ "pos_z": 99.28125,
+ "stations": "Narbeth's Progress,Szilard Beacon,Ampere Landing"
+ },
+ {
+ "name": "Djiwar",
+ "pos_x": -8.90625,
+ "pos_y": -33.6875,
+ "pos_z": 56.96875,
+ "stations": "Ride Installation,Roberts Orbital,Volta Lab,Szilard Platform"
+ },
+ {
+ "name": "Djowen",
+ "pos_x": 57.03125,
+ "pos_y": -203.34375,
+ "pos_z": -44.59375,
+ "stations": "Plante Bastion,Harawi Point"
+ },
+ {
+ "name": "Djowenet",
+ "pos_x": 37.6875,
+ "pos_y": -23.96875,
+ "pos_z": 117,
+ "stations": "Cavendish Hub,Meikle Dock,Zhen Gateway,Foucault Ring,Parazynski Port,Lichtenberg Dock,Adamson Dock,Barnaby Laboratory,Volterra Vista,Eudoxus Installation,Howard Survey,Holland Beacon,Siegel Holdings"
+ },
+ {
+ "name": "Djuhti",
+ "pos_x": -6.8125,
+ "pos_y": 92.65625,
+ "pos_z": 24.8125,
+ "stations": "Brunner Colony,Lawson Camp,Morgan Installation"
+ },
+ {
+ "name": "Djuhty",
+ "pos_x": -17.84375,
+ "pos_y": -131.375,
+ "pos_z": -32.1875,
+ "stations": "Harding Settlement"
+ },
+ {
+ "name": "DK Leonis",
+ "pos_x": 24.90625,
+ "pos_y": 61.15625,
+ "pos_z": -36.1875,
+ "stations": "Leavitt Plant,Wingqvist Dock,Newcomen Orbital,Barjavel Landing"
+ },
+ {
+ "name": "DK Ursae Majoris",
+ "pos_x": -48.96875,
+ "pos_y": 66.03125,
+ "pos_z": -63.9375,
+ "stations": "Slusser Hub,Csoma Landing,Westerfeld City"
+ },
+ {
+ "name": "Dobunn",
+ "pos_x": -55.25,
+ "pos_y": 5.25,
+ "pos_z": 118.5625,
+ "stations": "Robinson Enterprise,Landis' Folly,Serre Enterprise"
+ },
+ {
+ "name": "Docleachi",
+ "pos_x": 76.96875,
+ "pos_y": -2.9375,
+ "pos_z": 21.15625,
+ "stations": "Anthony Landing,al-Haytham Station,Herndon Settlement,Shosuke Depot,Kroehl Vision"
+ },
+ {
+ "name": "Dogoneja",
+ "pos_x": 77.46875,
+ "pos_y": -1.125,
+ "pos_z": 119.78125,
+ "stations": "Maire Vision,Midgley's Progress,Baker Platform"
+ },
+ {
+ "name": "Dohkwibur",
+ "pos_x": 109.71875,
+ "pos_y": 16.875,
+ "pos_z": -95.96875,
+ "stations": "Saberhagen Mine,von Bellingshausen Outpost"
+ },
+ {
+ "name": "Dohkwithi",
+ "pos_x": -63.34375,
+ "pos_y": 148.21875,
+ "pos_z": 10.25,
+ "stations": "Ron Hubbard Dock,Barnaby Orbital"
+ },
+ {
+ "name": "Dolanque",
+ "pos_x": 109.15625,
+ "pos_y": 65.28125,
+ "pos_z": -3.1875,
+ "stations": "Bolotov Outpost,Lamarck Dock,Barr Relay"
+ },
+ {
+ "name": "Domawilata",
+ "pos_x": 100.6875,
+ "pos_y": -120.3125,
+ "pos_z": 142.15625,
+ "stations": "Bohr Retreat"
+ },
+ {
+ "name": "Domocast",
+ "pos_x": 41.6875,
+ "pos_y": -134.03125,
+ "pos_z": 129.28125,
+ "stations": "Yerka Dock,Okorafor Prospect,Yuzhe's Claim"
+ },
+ {
+ "name": "Domoira",
+ "pos_x": 10.03125,
+ "pos_y": 111.125,
+ "pos_z": -83.21875,
+ "stations": "Staden Dock,Heaviside Dock,Andree Dock,Quaglia Settlement,Hadamard's Progress"
+ },
+ {
+ "name": "Domoirene",
+ "pos_x": -22.96875,
+ "pos_y": 79.75,
+ "pos_z": 41.84375,
+ "stations": "Bulychev Station,Lasswitz Hub,Low Hub,Walter Silo,Mitropoulos Orbital,Lee Terminal,Cochrane Mine"
+ },
+ {
+ "name": "Domoth",
+ "pos_x": 33.0625,
+ "pos_y": -127,
+ "pos_z": -42.46875,
+ "stations": "van den Bergh Hub,Rondon Plant,Hague Port,Fujikawa Dock,Ross Holdings"
+ },
+ {
+ "name": "Domotwa",
+ "pos_x": 47,
+ "pos_y": 42.96875,
+ "pos_z": 23.65625,
+ "stations": "Coke Dock"
+ },
+ {
+ "name": "Domovoi",
+ "pos_x": -44,
+ "pos_y": 80.25,
+ "pos_z": -83.21875,
+ "stations": "Vaez de Torres Stop"
+ },
+ {
+ "name": "Don",
+ "pos_x": -38.125,
+ "pos_y": -51.78125,
+ "pos_z": 28.53125,
+ "stations": "Solovyov City,Alcala Station,Chapman City"
+ },
+ {
+ "name": "DON 680",
+ "pos_x": 57.71875,
+ "pos_y": -10.34375,
+ "pos_z": 56.3125,
+ "stations": "Bouch Port,Shukor Terminal"
+ },
+ {
+ "name": "Dong Ku",
+ "pos_x": 10.34375,
+ "pos_y": -4.375,
+ "pos_z": 138.03125,
+ "stations": "Priest Settlement"
+ },
+ {
+ "name": "Dong Wang",
+ "pos_x": 57.34375,
+ "pos_y": -121,
+ "pos_z": 140.78125,
+ "stations": "Goodman Vision"
+ },
+ {
+ "name": "Dongasa",
+ "pos_x": 110.25,
+ "pos_y": -26.875,
+ "pos_z": 91.75,
+ "stations": "Dawson Enterprise,Leberecht Tempel Port,Froud Holdings,McMillan Terminal,Beaumont Base"
+ },
+ {
+ "name": "Dongat",
+ "pos_x": 101.75,
+ "pos_y": -161.8125,
+ "pos_z": 140.59375,
+ "stations": "Kotelnikov Terminal,Riebe Landing,Oren Survey"
+ },
+ {
+ "name": "Dongkum",
+ "pos_x": -17.875,
+ "pos_y": -11.75,
+ "pos_z": -90.4375,
+ "stations": "Hahn Ring,Swanwick Enterprise,Back Port,Alten City,Kotzebue Laboratory,Waldrop Enterprise,Sagan Port"
+ },
+ {
+ "name": "Donglavs",
+ "pos_x": -28.9375,
+ "pos_y": -179.375,
+ "pos_z": -22.8125,
+ "stations": "Hiyya Orbital,Cartan Installation,Maupertuis Survey"
+ },
+ {
+ "name": "Dongones",
+ "pos_x": 77.78125,
+ "pos_y": -178.78125,
+ "pos_z": 4.28125,
+ "stations": "Woodroffe Installation,August von Steinheil Hub,Galois Vision,al-Kashi Terminal"
+ },
+ {
+ "name": "Dongzi",
+ "pos_x": 61.65625,
+ "pos_y": -93.71875,
+ "pos_z": -14.03125,
+ "stations": "Penrose Ring,Farmer Reach"
+ },
+ {
+ "name": "Doolona",
+ "pos_x": 114.28125,
+ "pos_y": -166.40625,
+ "pos_z": 108.1875,
+ "stations": "Hughes Vision,Vaucouleurs Station,Arago Vision"
+ },
+ {
+ "name": "Doolonii",
+ "pos_x": -30.96875,
+ "pos_y": -73.75,
+ "pos_z": 174.90625,
+ "stations": "Nielsen Arena"
+ },
+ {
+ "name": "Doquiatec",
+ "pos_x": 136.90625,
+ "pos_y": -64.1875,
+ "pos_z": -81.875,
+ "stations": "Wu Enterprise,Fieseler Dock"
+ },
+ {
+ "name": "Doris",
+ "pos_x": -38.875,
+ "pos_y": 69.03125,
+ "pos_z": -13.375,
+ "stations": "Beebe Dock,Carlisle Refinery,Bulmer Terminal,Tapinas Terminal"
+ },
+ {
+ "name": "Dount",
+ "pos_x": 153.96875,
+ "pos_y": -43.28125,
+ "pos_z": -42,
+ "stations": "Bohme Vision,Peirce Station,Airy Refinery,Bates Orbital"
+ },
+ {
+ "name": "Dounthiassi",
+ "pos_x": 107.59375,
+ "pos_y": -156,
+ "pos_z": 78.625,
+ "stations": "Weaver Ring,Mattingly Vision,Grushin Orbital,d'Arrest Horizons,Webb Depot,Gallun Settlement"
+ },
+ {
+ "name": "Dountidi",
+ "pos_x": 103.90625,
+ "pos_y": 4.625,
+ "pos_z": 19.78125,
+ "stations": "White Gateway,Hume Enterprise"
+ },
+ {
+ "name": "DP Camelopardalis",
+ "pos_x": -50.875,
+ "pos_y": 20.21875,
+ "pos_z": -76.96875,
+ "stations": "Zewail Dock"
+ },
+ {
+ "name": "DR Crucis",
+ "pos_x": 83.25,
+ "pos_y": 8.03125,
+ "pos_z": 53.34375,
+ "stations": "Harness Settlement,Narvaez Arsenal,Shirley Platform"
+ },
+ {
+ "name": "Dragese",
+ "pos_x": -33.34375,
+ "pos_y": -113.1875,
+ "pos_z": 90.25,
+ "stations": "Anderson Prospect,Bowen Port,O'Brien's Progress"
+ },
+ {
+ "name": "Draguan Nu",
+ "pos_x": -70.0625,
+ "pos_y": -81.875,
+ "pos_z": -73.03125,
+ "stations": "Reightler Dock,Smith Depot,Qureshi Landing"
+ },
+ {
+ "name": "Draguti",
+ "pos_x": 42.40625,
+ "pos_y": -174.375,
+ "pos_z": 154.28125,
+ "stations": "Sorayama Base"
+ },
+ {
+ "name": "Draualang",
+ "pos_x": 91.1875,
+ "pos_y": 65.15625,
+ "pos_z": -38.40625,
+ "stations": "Otiman Colony"
+ },
+ {
+ "name": "Draudirawah",
+ "pos_x": 199.3125,
+ "pos_y": -121.375,
+ "pos_z": 62,
+ "stations": "Cayley Dock,Helffrich Oasis,Lyne Terminal,Steele Beacon"
+ },
+ {
+ "name": "Draunetes",
+ "pos_x": -39.71875,
+ "pos_y": -77.3125,
+ "pos_z": -141.6875,
+ "stations": "Andreas Camp"
+ },
+ {
+ "name": "Draur",
+ "pos_x": -69.625,
+ "pos_y": -7.4375,
+ "pos_z": 150.5625,
+ "stations": "Creighton Terminal,Goulart Point,Beatty Beacon"
+ },
+ {
+ "name": "Dread",
+ "pos_x": -44.65625,
+ "pos_y": -86.5625,
+ "pos_z": 13.03125,
+ "stations": "Luiken Hub,Ingstad City,von Bellingshausen Port,Baydukov Landing,Burton Enterprise"
+ },
+ {
+ "name": "Drevelli",
+ "pos_x": 110.4375,
+ "pos_y": 27.84375,
+ "pos_z": -138.15625,
+ "stations": "Wyndham Depot"
+ },
+ {
+ "name": "Drevlya Mu",
+ "pos_x": -142.03125,
+ "pos_y": -112.53125,
+ "pos_z": -18.65625,
+ "stations": "Griffith Landing,Stuart Settlement,Charnas Terminal"
+ },
+ {
+ "name": "Drevlyada",
+ "pos_x": -40.90625,
+ "pos_y": -35.4375,
+ "pos_z": 86.34375,
+ "stations": "Grandin Port,Chamitoff Dock,Hermaszewski Terminal,Berners-Lee Ring,Salam Station,Jacobi Holdings,Wheeler Dock,Bagian City,Dukaj Works,Borman Terminal,Aleksandrov City"
+ },
+ {
+ "name": "Drevlyan",
+ "pos_x": -121.1875,
+ "pos_y": 49.3125,
+ "pos_z": -123.75,
+ "stations": "Lopez de Legazpi Mines,Wingrove Depot,Pierce Landing"
+ },
+ {
+ "name": "DS Leonis",
+ "pos_x": 10.25,
+ "pos_y": 34.875,
+ "pos_z": -12.34375,
+ "stations": "Moore Installation,Grandin Terminal"
+ },
+ {
+ "name": "DT Virginis",
+ "pos_x": 6.9375,
+ "pos_y": 35.03125,
+ "pos_z": 6.21875,
+ "stations": "So-yeon Vision"
+ },
+ {
+ "name": "Du Shama",
+ "pos_x": -64.78125,
+ "pos_y": 8.28125,
+ "pos_z": 82.625,
+ "stations": "Needham Platform,Vaugh Landing"
+ },
+ {
+ "name": "Du Shamitra",
+ "pos_x": 78.4375,
+ "pos_y": -102.53125,
+ "pos_z": 80.84375,
+ "stations": "Hartmann Platform,Molchanov Survey"
+ },
+ {
+ "name": "Du Shas",
+ "pos_x": 141.84375,
+ "pos_y": -87.375,
+ "pos_z": 21.4375,
+ "stations": "Goldreich Orbital,Bullialdus Mines,Ikeya Hub,Schiaparelli Depot"
+ },
+ {
+ "name": "Du Shenge",
+ "pos_x": -43.5625,
+ "pos_y": 17.3125,
+ "pos_z": -69.84375,
+ "stations": "Rosenberg Plant,Moore Orbital,Brackett Port,Volterra Settlement"
+ },
+ {
+ "name": "Duamta",
+ "pos_x": 2.1875,
+ "pos_y": 6.625,
+ "pos_z": -7,
+ "stations": "Wang City,Davis Terminal,Hurston Arsenal,Polyakov Station,Amis Installation,Herrington City,Vries Base,Walz Base,Stuart Prospect,Vesalius City"
+ },
+ {
+ "name": "Dubbuennel",
+ "pos_x": -9521.40625,
+ "pos_y": -913.5625,
+ "pos_z": 19786.53125,
+ "stations": "Dunker's Rest"
+ },
+ {
+ "name": "Duberdicus",
+ "pos_x": 21.375,
+ "pos_y": -85,
+ "pos_z": 47.25,
+ "stations": "Foster Hub,Bond Park,Bartini Dock"
+ },
+ {
+ "name": "Dubhe",
+ "pos_x": -46.46875,
+ "pos_y": 96.09375,
+ "pos_z": -60.9375,
+ "stations": "Chargaff Orbital,Deere Point,Vonnegut Terminal"
+ },
+ {
+ "name": "Dubiaku",
+ "pos_x": 82.4375,
+ "pos_y": 120.0625,
+ "pos_z": 83.375,
+ "stations": "Auld Point"
+ },
+ {
+ "name": "Duc Babal",
+ "pos_x": 143.375,
+ "pos_y": -192.375,
+ "pos_z": -31.96875,
+ "stations": "Delaunay Relay"
+ },
+ {
+ "name": "Duc Bari",
+ "pos_x": 38.375,
+ "pos_y": 108.03125,
+ "pos_z": -55.28125,
+ "stations": "Rushd City,Boulton Holdings"
+ },
+ {
+ "name": "Ducat",
+ "pos_x": 43.5,
+ "pos_y": 9.59375,
+ "pos_z": -80.9375,
+ "stations": "Napier Base,Khrenov Beacon,Lee Platform"
+ },
+ {
+ "name": "Dudugara",
+ "pos_x": -107.8125,
+ "pos_y": -59.21875,
+ "pos_z": -101.96875,
+ "stations": "Lucid Camp"
+ },
+ {
+ "name": "Duduninke",
+ "pos_x": 77.625,
+ "pos_y": 57.84375,
+ "pos_z": 118.1875,
+ "stations": "Archambault Outpost,Garay Platform"
+ },
+ {
+ "name": "Duduseklis",
+ "pos_x": 48.375,
+ "pos_y": -78.34375,
+ "pos_z": -16.40625,
+ "stations": "Kuiper Plant,Giacobini Horizons,Roche Port"
+ },
+ {
+ "name": "Dugnais",
+ "pos_x": 145.125,
+ "pos_y": -99.96875,
+ "pos_z": -48.65625,
+ "stations": "Maury Orbital,van Vogt Lab,Gray Holdings"
+ },
+ {
+ "name": "Dugnates",
+ "pos_x": -2.78125,
+ "pos_y": 35.65625,
+ "pos_z": 117.71875,
+ "stations": "Moresby Settlement,Chiang Installation,Clapperton Settlement"
+ },
+ {
+ "name": "Dugnatlehi",
+ "pos_x": 75.34375,
+ "pos_y": -204.625,
+ "pos_z": -61.75,
+ "stations": "Nadiradze Vision,Davidson Installation,Dishoeck Station,Plaskett Hub"
+ },
+ {
+ "name": "Dulerce",
+ "pos_x": 49.03125,
+ "pos_y": 25.6875,
+ "pos_z": -73,
+ "stations": "Wiener Terminal,Kazantsev Dock,Scithers City,Hippalus Hub,Harper Terminal,Minkowski Works,Kettle Vision"
+ },
+ {
+ "name": "Dulla",
+ "pos_x": -7.28125,
+ "pos_y": 60.4375,
+ "pos_z": 60.625,
+ "stations": "Lee Colony"
+ },
+ {
+ "name": "Dulos",
+ "pos_x": 29,
+ "pos_y": -71.34375,
+ "pos_z": 45.5,
+ "stations": "Milnor Station,Wilson Ring,Smith Port,Asire Vision,Carpenter's Claim,Regiomontanus Vision,Bohnhoff Dock,Saunders City,Quimby's Inheritance"
+ },
+ {
+ "name": "Duloskitoke",
+ "pos_x": 90.125,
+ "pos_y": -38.46875,
+ "pos_z": -3.96875,
+ "stations": "Lawrence Orbital,Moisuc Enterprise"
+ },
+ {
+ "name": "Dumni",
+ "pos_x": 22.5,
+ "pos_y": -198.8125,
+ "pos_z": -42,
+ "stations": "Crown Works"
+ },
+ {
+ "name": "Dumnites",
+ "pos_x": 138.21875,
+ "pos_y": -120.25,
+ "pos_z": -22.0625,
+ "stations": "Whitney City,Chernykh Installation,Jekhowsky Orbital,Araki Station,Albumasar City,McDermott Dock,Naubakht Ring,Britnev Settlement"
+ },
+ {
+ "name": "Dumnon",
+ "pos_x": -0.5625,
+ "pos_y": -17.375,
+ "pos_z": 107.03125,
+ "stations": "Melvill Horizons,Illy Dock"
+ },
+ {
+ "name": "Dumnona",
+ "pos_x": -16.4375,
+ "pos_y": 4.78125,
+ "pos_z": 52.65625,
+ "stations": "Ricci Port,Dias Port,Koontz's Progress,Bowen Bastion,Perrin Enterprise"
+ },
+ {
+ "name": "Durgeth",
+ "pos_x": 109.8125,
+ "pos_y": -122.0625,
+ "pos_z": 126.4375,
+ "stations": "Abetti Mines,Mies van der Rohe Landing"
+ },
+ {
+ "name": "Durinn",
+ "pos_x": 6.96875,
+ "pos_y": 66.75,
+ "pos_z": -73.5625,
+ "stations": "Converse Platform"
+ },
+ {
+ "name": "Durius",
+ "pos_x": -5.375,
+ "pos_y": -86.3125,
+ "pos_z": 52.75,
+ "stations": "Pimi Orbital,Nilson's Pride,Sikorsky Penal colony"
+ },
+ {
+ "name": "Duro",
+ "pos_x": 117.34375,
+ "pos_y": -119.0625,
+ "pos_z": 153,
+ "stations": "Abetti Vision,Haber Point,Tupolev Terminal,Wheeler Station,McKay Refinery"
+ },
+ {
+ "name": "Durojin",
+ "pos_x": -83.53125,
+ "pos_y": -18.9375,
+ "pos_z": 73.25,
+ "stations": "Lavrador Platform,Reis Port,Panshin Enterprise,Moran Landing"
+ },
+ {
+ "name": "Durok",
+ "pos_x": -99.3125,
+ "pos_y": -43.03125,
+ "pos_z": 118.9375,
+ "stations": "Zettel Colony,Lasswitz Landing,Baxter Mines,Barnes Arsenal"
+ },
+ {
+ "name": "Duronese",
+ "pos_x": 66.5625,
+ "pos_y": -146.4375,
+ "pos_z": 1.59375,
+ "stations": "Mukai Platform,Mohr Vision"
+ },
+ {
+ "name": "Duroua",
+ "pos_x": 46.0625,
+ "pos_y": -183.40625,
+ "pos_z": 81.9375,
+ "stations": "Mrkos Outpost"
+ },
+ {
+ "name": "Durya Po",
+ "pos_x": 69.5625,
+ "pos_y": 108.59375,
+ "pos_z": 28.375,
+ "stations": "Rotsler Beacon,Herodotus Enterprise,Harvey Arsenal,Binder Prospect"
+ },
+ {
+ "name": "Duryampas",
+ "pos_x": 99.78125,
+ "pos_y": -103.1875,
+ "pos_z": 121.90625,
+ "stations": "van de Hulst Station,Marcy Dock,Brashear Station,Napier Keep"
+ },
+ {
+ "name": "Duulngandi",
+ "pos_x": 19.21875,
+ "pos_y": 158.46875,
+ "pos_z": 4.53125,
+ "stations": "Davy Camp"
+ },
+ {
+ "name": "Duulngari",
+ "pos_x": 175.65625,
+ "pos_y": -106.78125,
+ "pos_z": 5.15625,
+ "stations": "Grushin Orbital,Lilienthal Orbital"
+ },
+ {
+ "name": "Duwali",
+ "pos_x": -96.09375,
+ "pos_y": 30.15625,
+ "pos_z": 19.71875,
+ "stations": "Bisson Dock,Pratchett Orbital,Hume Orbital,Scalzi's Folly,Bova Terminal"
+ },
+ {
+ "name": "Duwar",
+ "pos_x": -141.03125,
+ "pos_y": -57.125,
+ "pos_z": 87,
+ "stations": "Junlong Landing,Mayer Terminal,Soto Beacon"
+ },
+ {
+ "name": "Duwaredari",
+ "pos_x": 62.8125,
+ "pos_y": -52,
+ "pos_z": -1.5625,
+ "stations": "Scott Dock,Gehrels Refinery,Garrett Dock,Oosterhoff Vista"
+ },
+ {
+ "name": "Dvorotri",
+ "pos_x": -129.625,
+ "pos_y": 39.40625,
+ "pos_z": -91.28125,
+ "stations": "Lyell's Progress,Lovell Colony,Khan Settlement"
+ },
+ {
+ "name": "Dvorsi",
+ "pos_x": 77.59375,
+ "pos_y": -96.5625,
+ "pos_z": 7.3125,
+ "stations": "Fowler Orbital,Severin Terminal,Chernykh Ring,Alfven Prospect,Qureshi Vision,Schwarzschild Port,Dowty Terminal"
+ },
+ {
+ "name": "Dvoruba",
+ "pos_x": 9.5625,
+ "pos_y": -1.78125,
+ "pos_z": -34.28125,
+ "stations": "Whitworth Dock,Snyder Settlement"
+ },
+ {
+ "name": "DW Camelopardalis",
+ "pos_x": -62.625,
+ "pos_y": 34.8125,
+ "pos_z": -85.8125,
+ "stations": "Haxel Stop,Casper Terminal"
+ },
+ {
+ "name": "DX 799",
+ "pos_x": -22.96875,
+ "pos_y": 12.3125,
+ "pos_z": -17.90625,
+ "stations": "Malenchenko Penal colony,Feoktistov Colony"
+ },
+ {
+ "name": "Dxui",
+ "pos_x": 97.375,
+ "pos_y": -160.375,
+ "pos_z": 11.875,
+ "stations": "Huggins Port,Moffat Park,Elmore Hub,Nojiri Hub"
+ },
+ {
+ "name": "Dyaus",
+ "pos_x": 41.09375,
+ "pos_y": -182.6875,
+ "pos_z": 4.78125,
+ "stations": "Kirkwood Relay"
+ },
+ {
+ "name": "Dyaushibi",
+ "pos_x": 112.875,
+ "pos_y": -20.75,
+ "pos_z": -33.03125,
+ "stations": "Curie Port,Boas Terminal,Carpenter Enterprise,Vaugh Market,Elion Enterprise,Hawking Hub,Griffin Relay,Melvill Relay,Howe Port"
+ },
+ {
+ "name": "Dyava",
+ "pos_x": 132.71875,
+ "pos_y": -18.09375,
+ "pos_z": -101.5625,
+ "stations": "Ellis Enterprise,Lopez-Alegria Platform,Edison Landing,Morrison Survey,Lasswitz Prospect"
+ },
+ {
+ "name": "Dyavansana",
+ "pos_x": -80.375,
+ "pos_y": 147.59375,
+ "pos_z": 19.71875,
+ "stations": "Drew Settlement,Bowen Beacon,Helmholtz Station"
+ },
+ {
+ "name": "Dyavareldi",
+ "pos_x": 123.90625,
+ "pos_y": -11.6875,
+ "pos_z": 80.09375,
+ "stations": "Brooks Dock,Roe Dock,Bothezat Ring,Mohr Prospect,Hill Plant"
+ },
+ {
+ "name": "Dyavata",
+ "pos_x": 24.78125,
+ "pos_y": 19.75,
+ "pos_z": 116.53125,
+ "stations": "Landsteiner Works,Massimino Plant"
+ },
+ {
+ "name": "Dyestla",
+ "pos_x": 109.375,
+ "pos_y": -143.34375,
+ "pos_z": 65.46875,
+ "stations": "Nakano Orbital,McKie Landing,Clark Survey"
+ },
+ {
+ "name": "Dyin",
+ "pos_x": 35.0625,
+ "pos_y": -175.5625,
+ "pos_z": -2.15625,
+ "stations": "Backlund Hub"
+ },
+ {
+ "name": "Dyindumasci",
+ "pos_x": 83.15625,
+ "pos_y": -50.21875,
+ "pos_z": -51.65625,
+ "stations": "Bernoulli Hub,Cole Terminal"
+ },
+ {
+ "name": "Dyingia",
+ "pos_x": -6.625,
+ "pos_y": -163.5,
+ "pos_z": -80.875,
+ "stations": "Buckell Colony,Felice Port,Normand Enterprise"
+ },
+ {
+ "name": "Dyinyinga",
+ "pos_x": 138.6875,
+ "pos_y": -104.1875,
+ "pos_z": 79.0625,
+ "stations": "Lassell Works"
+ },
+ {
+ "name": "Dyr",
+ "pos_x": 90.71875,
+ "pos_y": -24.75,
+ "pos_z": -7.5,
+ "stations": "Archambault Port"
+ },
+ {
+ "name": "Dzacab",
+ "pos_x": 108.53125,
+ "pos_y": 52.78125,
+ "pos_z": 60.78125,
+ "stations": "Weber Works,Holub Base,Rubruck Depot"
+ },
+ {
+ "name": "DzacaliTem",
+ "pos_x": 104.5,
+ "pos_y": -110.9375,
+ "pos_z": -83.09375,
+ "stations": "Bartini Terminal,Zhuravleva Orbital,Baracchi Hub,Shipton Camp"
+ },
+ {
+ "name": "Dzacamaich",
+ "pos_x": 93.65625,
+ "pos_y": -167.6875,
+ "pos_z": 122.5625,
+ "stations": "Horrocks Mines,Alexander Station,Foster Keep"
+ },
+ {
+ "name": "Dzacani",
+ "pos_x": -30.28125,
+ "pos_y": -126.21875,
+ "pos_z": 68.8125,
+ "stations": "Berkey Vision,Seitter Landing,Hanke-Woods Dock"
+ },
+ {
+ "name": "Dzacara",
+ "pos_x": 116.3125,
+ "pos_y": -137.21875,
+ "pos_z": -21.5625,
+ "stations": "Borrego Terminal,Whitford Works,Juan de la Cierva Point"
+ },
+ {
+ "name": "Dzalak Mani",
+ "pos_x": 98.90625,
+ "pos_y": -191.28125,
+ "pos_z": 110.625,
+ "stations": "Mueller Station,Reed Camp,Schiaparelli Gateway,Speke Arsenal"
+ },
+ {
+ "name": "DzaliTemu",
+ "pos_x": 79.46875,
+ "pos_y": -18.8125,
+ "pos_z": 20.8125,
+ "stations": "Green Escape,Perrin Dock,Lambert Bastion"
+ },
+ {
+ "name": "Dziewong Bo",
+ "pos_x": 103.1875,
+ "pos_y": 117.96875,
+ "pos_z": -19.46875,
+ "stations": "Kennicott Oasis,Russell Prospect,Patterson's Inheritance"
+ },
+ {
+ "name": "Dziva",
+ "pos_x": -99.09375,
+ "pos_y": 105.09375,
+ "pos_z": 46.03125,
+ "stations": "Tudela Beacon,Friesner Survey"
+ },
+ {
+ "name": "Dzivaruba",
+ "pos_x": 170.59375,
+ "pos_y": -26.4375,
+ "pos_z": 57.3125,
+ "stations": "Bulychev Relay,Swanwick Terminal"
+ },
+ {
+ "name": "Dzivatae",
+ "pos_x": 118,
+ "pos_y": -99.5625,
+ "pos_z": -39.75,
+ "stations": "Bigourdan Landing"
+ },
+ {
+ "name": "Dzoavit",
+ "pos_x": 130.84375,
+ "pos_y": -9.6875,
+ "pos_z": 56.40625,
+ "stations": "Marlowe's Claim"
+ },
+ {
+ "name": "e Geminorum",
+ "pos_x": 33.65625,
+ "pos_y": 11.5,
+ "pos_z": -83.90625,
+ "stations": "Macgregor's Pride"
+ },
+ {
+ "name": "Eagle Sector IR-W d1-105",
+ "pos_x": -2046.21875,
+ "pos_y": 104.40625,
+ "pos_z": 6699.90625,
+ "stations": "Eagle Sector Secure Facility"
+ },
+ {
+ "name": "Eagle Sector IR-W d1-117",
+ "pos_x": -2054.09375,
+ "pos_y": 85.71875,
+ "pos_z": 6710.875,
+ "stations": "Eagle's Landing"
+ },
+ {
+ "name": "Earth Expeditionary Fleet",
+ "pos_x": -9552.0625,
+ "pos_y": -887.875,
+ "pos_z": 19834.1875,
+ "stations": "Vicktore's Promise"
+ },
+ {
+ "name": "Eban",
+ "pos_x": 146.96875,
+ "pos_y": -61.8125,
+ "pos_z": 72.8125,
+ "stations": "Lunney Vision,Henderson Prospect"
+ },
+ {
+ "name": "Ebana",
+ "pos_x": 151.65625,
+ "pos_y": -14.9375,
+ "pos_z": -15.03125,
+ "stations": "Caillie Hub,Baudin Enterprise,Biggle Dock,Shepherd Hub,Lenthall Beacon"
+ },
+ {
+ "name": "Ebandii",
+ "pos_x": 124.5625,
+ "pos_y": -101.28125,
+ "pos_z": -67.9375,
+ "stations": "Ohain Installation"
+ },
+ {
+ "name": "Ebanji",
+ "pos_x": -25.46875,
+ "pos_y": 87.75,
+ "pos_z": -129.65625,
+ "stations": "Fernandez Works,Williamson Hangar,Smith Landing,Raleigh Relay"
+ },
+ {
+ "name": "Ebisopiac",
+ "pos_x": -93.40625,
+ "pos_y": -110.15625,
+ "pos_z": 85.96875,
+ "stations": "Engle Works,Smith's Claim,Caryanda Beacon"
+ },
+ {
+ "name": "Ebisu",
+ "pos_x": 69.6875,
+ "pos_y": -78.3125,
+ "pos_z": -130.9375,
+ "stations": "Dietz Terminal,Cartmill Gateway,Vaez de Torres Hub,Cartan Survey,Roggeveen Installation"
+ },
+ {
+ "name": "Ebor",
+ "pos_x": -30.71875,
+ "pos_y": -49.6875,
+ "pos_z": -113.8125,
+ "stations": "Morris Enterprise,Santarem's Folly,Pierce Terminal,Goulart Gateway,Palmer Port,Ebor Xenobiological Facility"
+ },
+ {
+ "name": "Eborai",
+ "pos_x": 7.25,
+ "pos_y": -32.40625,
+ "pos_z": 116.5625,
+ "stations": "Sinclair Relay"
+ },
+ {
+ "name": "Eburnakura",
+ "pos_x": 92.375,
+ "pos_y": -85.21875,
+ "pos_z": 66.65625,
+ "stations": "Parkinson Terminal,Koontz's Inheritance,Anderson Terminal"
+ },
+ {
+ "name": "Eburnatiate",
+ "pos_x": -26.53125,
+ "pos_y": -136.96875,
+ "pos_z": -32.03125,
+ "stations": "Clark Platform"
+ },
+ {
+ "name": "Edanditis",
+ "pos_x": 71.65625,
+ "pos_y": -33.6875,
+ "pos_z": 72.3125,
+ "stations": "Wisoff Port,Nelson Terminal,Lanchester Barracks,Euclid Hub,Pauling Settlement"
+ },
+ {
+ "name": "Ededleen",
+ "pos_x": 93.0625,
+ "pos_y": 41.4375,
+ "pos_z": 82.3125,
+ "stations": "Shaw Gateway,Tepper Hub,McDaniel Dock,Disch Horizons,Cortes Silo,Godel Ring,Monge Station,Tilley Vision,Quimper Forum"
+ },
+ {
+ "name": "Edenaiwan",
+ "pos_x": 115.5,
+ "pos_y": 101,
+ "pos_z": -13.875,
+ "stations": "Gemar Survey,Arber Relay,Kotov Landing"
+ },
+ {
+ "name": "Edenapel",
+ "pos_x": 6.1875,
+ "pos_y": 7.34375,
+ "pos_z": 39.21875,
+ "stations": "Lanier Station,Hennepin Station,Fuchs Port,Yu Market,Williams Prospect,Schilling Terminal,Lowry City,Kessel Hub,Sleator Port,Womack Hub,Meyrink Station,Potrykus City"
+ },
+ {
+ "name": "Edene",
+ "pos_x": 105.5,
+ "pos_y": 21.59375,
+ "pos_z": 109.9375,
+ "stations": "Wiley Enterprise,Montrose Hub,Mondeh Landing"
+ },
+ {
+ "name": "Edenses",
+ "pos_x": 14.46875,
+ "pos_y": -3.4375,
+ "pos_z": -104.375,
+ "stations": "Heng Gateway,Feynman Legacy"
+ },
+ {
+ "name": "Edensu",
+ "pos_x": 205.65625,
+ "pos_y": -166.28125,
+ "pos_z": 86.25,
+ "stations": "Graham Camp,Potocnik Dock"
+ },
+ {
+ "name": "Edenwi",
+ "pos_x": -50.75,
+ "pos_y": -169.84375,
+ "pos_z": -70.03125,
+ "stations": "Benyovszky Dock,Pinzon Beacon"
+ },
+ {
+ "name": "Edge Fraternity Landing",
+ "pos_x": -9544.5625,
+ "pos_y": -889.75,
+ "pos_z": 19810.5,
+ "stations": "Concordia Hub"
+ },
+ {
+ "name": "Edin",
+ "pos_x": 27.1875,
+ "pos_y": -43.96875,
+ "pos_z": -12.21875,
+ "stations": "Viehbock Dock"
+ },
+ {
+ "name": "Edindui",
+ "pos_x": -7.3125,
+ "pos_y": 56.75,
+ "pos_z": 98.8125,
+ "stations": "Abernathy Settlement"
+ },
+ {
+ "name": "Edinia",
+ "pos_x": -39.59375,
+ "pos_y": -38.09375,
+ "pos_z": 103.53125,
+ "stations": "Tiptree Plant"
+ },
+ {
+ "name": "Edinifex",
+ "pos_x": -35.5625,
+ "pos_y": 22.4375,
+ "pos_z": -142.8125,
+ "stations": "Popovich Dock,Quimper Depot,Velazquez Hub"
+ },
+ {
+ "name": "Edinura",
+ "pos_x": 128.53125,
+ "pos_y": -189.5625,
+ "pos_z": 100.96875,
+ "stations": "Anthony's Progress,Dixon Terminal,Wigura's Progress,Block Base"
+ },
+ {
+ "name": "Eea",
+ "pos_x": 12.125,
+ "pos_y": 3.15625,
+ "pos_z": 44.78125,
+ "stations": "Malocello Point,Bell Colony,Shriver Settlement"
+ },
+ {
+ "name": "Eeyen",
+ "pos_x": 101.5625,
+ "pos_y": 45.21875,
+ "pos_z": 22.59375,
+ "stations": "Bova Station,Erikson Hub,Baturin Landing"
+ },
+ {
+ "name": "Efnisei",
+ "pos_x": -113.5625,
+ "pos_y": 60.25,
+ "pos_z": 74.90625,
+ "stations": "Bobko Base,Stephens Landing,Potrykus Mine"
+ },
+ {
+ "name": "Ega",
+ "pos_x": 31.75,
+ "pos_y": 17.5625,
+ "pos_z": 114.09375,
+ "stations": "Metz Enterprise,Clauss Point"
+ },
+ {
+ "name": "Egeria",
+ "pos_x": 44.3125,
+ "pos_y": -31.625,
+ "pos_z": -22,
+ "stations": "Wyeth Station,Brosnan Arsenal,Acton Settlement"
+ },
+ {
+ "name": "EGGR 431",
+ "pos_x": 31.6875,
+ "pos_y": 36.75,
+ "pos_z": -28.40625,
+ "stations": "Weston Terminal,Jordan Survey,Brahe Ring"
+ },
+ {
+ "name": "EGM 559",
+ "pos_x": 0.1875,
+ "pos_y": -77.78125,
+ "pos_z": -3.6875,
+ "stations": "Burke Dock"
+ },
+ {
+ "name": "EGM 762",
+ "pos_x": 12.375,
+ "pos_y": -79.1875,
+ "pos_z": -3.59375,
+ "stations": "Neff Dock,la Cosa Survey"
+ },
+ {
+ "name": "EGM 823",
+ "pos_x": 10.59375,
+ "pos_y": -75.875,
+ "pos_z": -9.03125,
+ "stations": "Cabral Terminal,Stephens Works,Macan Dock,Velho Terminal"
+ },
+ {
+ "name": "Egovach",
+ "pos_x": -62.3125,
+ "pos_y": -72.0625,
+ "pos_z": -15.9375,
+ "stations": "Vernadsky Colony,Jett Mines"
+ },
+ {
+ "name": "Egovae",
+ "pos_x": 61.8125,
+ "pos_y": -79.125,
+ "pos_z": 32.9375,
+ "stations": "van den Bergh Orbital,Endate Market,Henslow Lab,Mason Arena,Biruni Silo"
+ },
+ {
+ "name": "Egovi",
+ "pos_x": 55.5,
+ "pos_y": -82.125,
+ "pos_z": 174.15625,
+ "stations": "Longyear Enterprise,Wafer Gateway,Keyes Terminal,Rowley Refinery,Neumann Palace"
+ },
+ {
+ "name": "Egunnlod",
+ "pos_x": 20.09375,
+ "pos_y": 123.9375,
+ "pos_z": -133.0625,
+ "stations": "Carpini Prospect"
+ },
+ {
+ "name": "Ehecatl",
+ "pos_x": 29.59375,
+ "pos_y": -42.25,
+ "pos_z": 42.125,
+ "stations": "Michelson Port,Liebig Station,Barratt Port,Atwater Gateway,Ivanishin Market,Hackworth Orbital,Sellers City,Planck Works,Cleave Depot,Volynov Gateway,Murdoch Dock"
+ },
+ {
+ "name": "Ehi",
+ "pos_x": 38.59375,
+ "pos_y": -33.625,
+ "pos_z": 18.21875,
+ "stations": "Mawson Holdings,Freud Colony,Jendrassik Mines"
+ },
+ {
+ "name": "Ehlanda",
+ "pos_x": 115.15625,
+ "pos_y": -160.8125,
+ "pos_z": 75.90625,
+ "stations": "Leopold Heckmann Port,Clark Port,Vavrova Station,Gottlob Frege Point"
+ },
+ {
+ "name": "Ehlangai",
+ "pos_x": -51.53125,
+ "pos_y": 35.15625,
+ "pos_z": -46.125,
+ "stations": "Slusser Station,Sekowski Station,Gilliland Point,Bulgarin Orbital,Nearchus Silo"
+ },
+ {
+ "name": "Ehlauneti",
+ "pos_x": -20.625,
+ "pos_y": -113.375,
+ "pos_z": -101.9375,
+ "stations": "Lee Orbital,O'Brien's Progress,Bellamy Installation"
+ },
+ {
+ "name": "Eingasan",
+ "pos_x": 130.09375,
+ "pos_y": -121.0625,
+ "pos_z": 98.75,
+ "stations": "Wachmann Vision,Flammarion Orbital,West Beacon,Zamyatin Base"
+ },
+ {
+ "name": "Eingin",
+ "pos_x": -45.625,
+ "pos_y": -79.5,
+ "pos_z": 94.40625,
+ "stations": "Drew Terminal,Hughes Base"
+ },
+ {
+ "name": "Einheriar",
+ "pos_x": -9557.8125,
+ "pos_y": -880.1875,
+ "pos_z": 19801.5625,
+ "stations": "Pilkington Orbital,Paxton Landing"
+ },
+ {
+ "name": "Eir",
+ "pos_x": 134.125,
+ "pos_y": 34.9375,
+ "pos_z": -42.125,
+ "stations": "Weyl Station,Jacobi Hub,Popper Landing,Jacobi Terminal"
+ },
+ {
+ "name": "Eitha",
+ "pos_x": 38.84375,
+ "pos_y": 10.5625,
+ "pos_z": 152.875,
+ "stations": "Mastracchio Hub,Song Gateway,Underwood Hub,Salk Town"
+ },
+ {
+ "name": "Eitha Di",
+ "pos_x": 79.75,
+ "pos_y": 28.53125,
+ "pos_z": -66.6875,
+ "stations": "Henslow Terminal,Ramon Plant,Sadi Carnot Terminal,Alvares Plant"
+ },
+ {
+ "name": "Eithure",
+ "pos_x": -82.53125,
+ "pos_y": 5.90625,
+ "pos_z": 115.78125,
+ "stations": "Stiegler Dock,Linaweaver Dock,Clair Dock"
+ },
+ {
+ "name": "Ejagalki",
+ "pos_x": 65.9375,
+ "pos_y": 47.53125,
+ "pos_z": 90.65625,
+ "stations": "Arkwright Base,Hudson Settlement,McCaffrey Point"
+ },
+ {
+ "name": "Ejagani",
+ "pos_x": -64.0625,
+ "pos_y": 117.0625,
+ "pos_z": 105.09375,
+ "stations": "Carpenter Platform,Alenquer Holdings"
+ },
+ {
+ "name": "Ejagarifuna",
+ "pos_x": 129.40625,
+ "pos_y": -139.0625,
+ "pos_z": 61.65625,
+ "stations": "Porubcan Port,Sopwith Depot,Mori Platform"
+ },
+ {
+ "name": "Ejagh",
+ "pos_x": -47.34375,
+ "pos_y": 48.40625,
+ "pos_z": 67.5,
+ "stations": "Treshchov Landing,Sarich Colony,Galilei Laboratory"
+ },
+ {
+ "name": "Ejaghaikul",
+ "pos_x": 4.75,
+ "pos_y": -73.3125,
+ "pos_z": 119.59375,
+ "stations": "Chretien Oudemans Terminal,Fehrenbach Terminal"
+ },
+ {
+ "name": "Ejagham",
+ "pos_x": 53.78125,
+ "pos_y": -20.375,
+ "pos_z": -23.15625,
+ "stations": "Glenn Ring,Henry Ring"
+ },
+ {
+ "name": "Ejaghan",
+ "pos_x": 113.28125,
+ "pos_y": 47.625,
+ "pos_z": 77.125,
+ "stations": "Mukai Enterprise,Joliot-Curie Port,Malpighi Enterprise,Alexandria Dock"
+ },
+ {
+ "name": "Ek Zi",
+ "pos_x": 128.6875,
+ "pos_y": 93.625,
+ "pos_z": 46.34375,
+ "stations": "Bass Relay,Thurston Beacon"
+ },
+ {
+ "name": "Ek Zi Gu",
+ "pos_x": 142.5625,
+ "pos_y": -84.71875,
+ "pos_z": -30.03125,
+ "stations": "Kempf Settlement"
+ },
+ {
+ "name": "Ek Ziyi",
+ "pos_x": -36.90625,
+ "pos_y": -177.84375,
+ "pos_z": 46.78125,
+ "stations": "Wendelin Camp,Gonnessiat Keep"
+ },
+ {
+ "name": "Ekchucosi",
+ "pos_x": 71.21875,
+ "pos_y": 114.1875,
+ "pos_z": 76.4375,
+ "stations": "Watts Dock,Bulgakov's Progress,Hyecho Dock,Rond d'Alembert Survey"
+ },
+ {
+ "name": "Ekhi",
+ "pos_x": -11.53125,
+ "pos_y": -34.6875,
+ "pos_z": -37.34375,
+ "stations": "Lost Henry,Ekhi Science Orbital,Busch Holdings"
+ },
+ {
+ "name": "Ekibiogaia",
+ "pos_x": -61.90625,
+ "pos_y": -120.4375,
+ "pos_z": 12.375,
+ "stations": "Cixin Station,Serrao Holdings,Mohun's Inheritance"
+ },
+ {
+ "name": "Ekibiogama",
+ "pos_x": 100.15625,
+ "pos_y": 84.375,
+ "pos_z": -85.03125,
+ "stations": "Collins Settlement"
+ },
+ {
+ "name": "Ekoimudji",
+ "pos_x": -42.625,
+ "pos_y": -143,
+ "pos_z": 18.59375,
+ "stations": "de Kamp Station,Hadid Hub,Kozin Holdings,Dickinson Vision,Coppel Beacon"
+ },
+ {
+ "name": "Ekoimunggu",
+ "pos_x": 83.375,
+ "pos_y": -16.0625,
+ "pos_z": 89.5625,
+ "stations": "Larbalestier Camp"
+ },
+ {
+ "name": "Ekoimurt",
+ "pos_x": 63.0625,
+ "pos_y": -68.875,
+ "pos_z": 80.53125,
+ "stations": "Block Hub,Bouwens Vision,Couper Orbital"
+ },
+ {
+ "name": "Ekondalie",
+ "pos_x": 43.125,
+ "pos_y": -22.9375,
+ "pos_z": -149.90625,
+ "stations": "Celebi's Pride,Steakley Observatory"
+ },
+ {
+ "name": "Ekondhri",
+ "pos_x": 110.71875,
+ "pos_y": -75.0625,
+ "pos_z": 112.5,
+ "stations": "Allen Gateway,Shipton Works,Holden Legacy"
+ },
+ {
+ "name": "Ekonii",
+ "pos_x": 28.625,
+ "pos_y": -236.71875,
+ "pos_z": 79.03125,
+ "stations": "Extra Landing"
+ },
+ {
+ "name": "Ekonir",
+ "pos_x": -37.78125,
+ "pos_y": 0.53125,
+ "pos_z": 63.90625,
+ "stations": "Morey Vision,Kubasov Orbital,Wiley Vision"
+ },
+ {
+ "name": "Ekono",
+ "pos_x": 59.125,
+ "pos_y": -155.15625,
+ "pos_z": 99.8125,
+ "stations": "Lundmark Terminal,Filippenko Survey,Bahcall Enterprise,Stasheff Camp"
+ },
+ {
+ "name": "Ekur",
+ "pos_x": -119.3125,
+ "pos_y": -52.875,
+ "pos_z": -56.15625,
+ "stations": "Duffy Orbital,Thomson City,Fontana Horizons,Harper Relay,Zahn Beacon"
+ },
+ {
+ "name": "Ekurninka",
+ "pos_x": -22.875,
+ "pos_y": 135.15625,
+ "pos_z": 59.28125,
+ "stations": "Morgan Dock,Nicholson Port,Nelder's Inheritance"
+ },
+ {
+ "name": "Ekuru",
+ "pos_x": -47.25,
+ "pos_y": -2.75,
+ "pos_z": 40.375,
+ "stations": "Dana Platform,Knight Plant,Lounge Holdings,Titov Vision"
+ },
+ {
+ "name": "El",
+ "pos_x": 23.625,
+ "pos_y": -13.0625,
+ "pos_z": -41.5,
+ "stations": "Titov Station,Volta Survey"
+ },
+ {
+ "name": "El Tio",
+ "pos_x": 36.96875,
+ "pos_y": -117.5625,
+ "pos_z": 25.4375,
+ "stations": "Kludze Dock,Rosenberg Base"
+ },
+ {
+ "name": "Elaba",
+ "pos_x": 86.84375,
+ "pos_y": -41.84375,
+ "pos_z": 118,
+ "stations": "Whitcomb Port,Hollander Hangar,Shklovsky Terminal,Khrenov Bastion"
+ },
+ {
+ "name": "Elata",
+ "pos_x": -82.875,
+ "pos_y": 93.09375,
+ "pos_z": -89.15625,
+ "stations": "Watts Horizons,Tilley Port,Shaara Installation,Bruce Point,Garrett Enterprise"
+ },
+ {
+ "name": "Elbiones",
+ "pos_x": 156,
+ "pos_y": -37.53125,
+ "pos_z": -39.90625,
+ "stations": "Lubbock Beacon"
+ },
+ {
+ "name": "Elboong",
+ "pos_x": -122.5,
+ "pos_y": -99.125,
+ "pos_z": 88.125,
+ "stations": "Spassky Port,Elcano Horizons,Grzimek Survey"
+ },
+ {
+ "name": "Elboongzi",
+ "pos_x": -122.5,
+ "pos_y": 48.34375,
+ "pos_z": -95,
+ "stations": "Alexandria Hub,Nicollier Settlement,Kondakova Port"
+ },
+ {
+ "name": "Electra",
+ "pos_x": -86.0625,
+ "pos_y": -159.9375,
+ "pos_z": -361.65625,
+ "stations": "Cavalieri,Rescue Ship - Cavalieri"
+ },
+ {
+ "name": "Eledas",
+ "pos_x": 153.15625,
+ "pos_y": 27.9375,
+ "pos_z": -9.53125,
+ "stations": "Searfoss Horizons,Payette Horizons,Howard Dock,J. G. Ballard's Progress"
+ },
+ {
+ "name": "Eledit",
+ "pos_x": -31.625,
+ "pos_y": -123.78125,
+ "pos_z": 25.6875,
+ "stations": "Lagerkvist Terminal"
+ },
+ {
+ "name": "Eledolyaks",
+ "pos_x": -66.40625,
+ "pos_y": 125.0625,
+ "pos_z": -17.875,
+ "stations": "Kimberlin Ring,Herreshoff Orbital,Fraser Platform,Hurston Settlement,Grego Penal colony"
+ },
+ {
+ "name": "Eledonga",
+ "pos_x": 99.125,
+ "pos_y": 18.28125,
+ "pos_z": 124.84375,
+ "stations": "Antonelli Depot,Zhen Platform"
+ },
+ {
+ "name": "Eleges",
+ "pos_x": 152.15625,
+ "pos_y": -71.59375,
+ "pos_z": 104.1875,
+ "stations": "Hertzsprung Prospect,Battani Colony,Resnik Hub,Miller Installation"
+ },
+ {
+ "name": "Eleu",
+ "pos_x": -29.65625,
+ "pos_y": 32.6875,
+ "pos_z": 104.84375,
+ "stations": "Finney Dock,Bao Orbital,Morris Works,Elder Station,Pierce Gateway,Bradshaw Station,Perrin Works,Tem Station,Farmer Silo,Qian Base,Chwedyk Base"
+ },
+ {
+ "name": "Eleumo",
+ "pos_x": 45.59375,
+ "pos_y": -59.0625,
+ "pos_z": 61.46875,
+ "stations": "Gurney Terminal,Gray Base,Villarceau Installation,Grigorovich Hub,Hildebrandt Penal colony"
+ },
+ {
+ "name": "Eleutsi",
+ "pos_x": 75.8125,
+ "pos_y": 17.78125,
+ "pos_z": 100.3125,
+ "stations": "Brule Retreat"
+ },
+ {
+ "name": "Elivagar",
+ "pos_x": -34.4375,
+ "pos_y": 21.5625,
+ "pos_z": -93.15625,
+ "stations": "Jett City,Savinykh Enterprise,Walker Terminal,Al-Jazari Dock,Gantt Gateway"
+ },
+ {
+ "name": "Elli",
+ "pos_x": 60.1875,
+ "pos_y": -1.03125,
+ "pos_z": -6.03125,
+ "stations": "Wright City,Baydukov Depot,Meikle Terminal,Ramelli Enterprise,Samokutyayev Reach"
+ },
+ {
+ "name": "Ellri",
+ "pos_x": 67.78125,
+ "pos_y": -42.28125,
+ "pos_z": 59.53125,
+ "stations": "Padalka Ring,DeLucas Enterprise,Johnson City,Hahn Enterprise"
+ },
+ {
+ "name": "Elska",
+ "pos_x": -63.875,
+ "pos_y": -10.78125,
+ "pos_z": -67.40625,
+ "stations": "Qurra Dock,Kuo Terminal,Faraday Point,Ferguson Dock"
+ },
+ {
+ "name": "Eluscap",
+ "pos_x": -30.03125,
+ "pos_y": 118.8125,
+ "pos_z": -0.65625,
+ "stations": "Fernandez Prospect,Jeschke Hub,Drake City,Crown Terminal"
+ },
+ {
+ "name": "Eluskapu",
+ "pos_x": -22.8125,
+ "pos_y": 29.1875,
+ "pos_z": -100.875,
+ "stations": "Miklouho-Maclay Settlement,Dummer Beacon"
+ },
+ {
+ "name": "Elusughna",
+ "pos_x": -69.28125,
+ "pos_y": -81.84375,
+ "pos_z": -3.90625,
+ "stations": "Hausdorff City,Waldrop City"
+ },
+ {
+ "name": "Elycoch",
+ "pos_x": 137.40625,
+ "pos_y": 57.0625,
+ "pos_z": 45.34375,
+ "stations": "Fearn Gateway"
+ },
+ {
+ "name": "Elycochos",
+ "pos_x": -71.4375,
+ "pos_y": 165.21875,
+ "pos_z": -15.1875,
+ "stations": "Borel Sanctuary"
+ },
+ {
+ "name": "Elycoco",
+ "pos_x": -18.84375,
+ "pos_y": -261.65625,
+ "pos_z": 15.71875,
+ "stations": "Stiles' Claim,Porges Survey,Robert Point"
+ },
+ {
+ "name": "Elysia",
+ "pos_x": 115.09375,
+ "pos_y": -110.53125,
+ "pos_z": 16.59375,
+ "stations": "Tavares Point,L.L.G. Ladra One,Tilley Holdings,Snodgrass Settlement"
+ },
+ {
+ "name": "Ember",
+ "pos_x": 64.21875,
+ "pos_y": -139.4375,
+ "pos_z": -23.03125,
+ "stations": "Lintott Terminal,van Houten Station,Reid Ring,Shaver's Folly"
+ },
+ {
+ "name": "Emblandjin",
+ "pos_x": 106.84375,
+ "pos_y": 70.0625,
+ "pos_z": 52.25,
+ "stations": "Gibson Gateway,Minkowski Station,Blaschke Point"
+ },
+ {
+ "name": "Emblannovii",
+ "pos_x": 67.53125,
+ "pos_y": -214,
+ "pos_z": 82.3125,
+ "stations": "Bouwens Colony,Fehrenbach Terminal,Gaensler Station,Tago Hub,Hussenot Orbital,Artsutanov Orbital,King Landing"
+ },
+ {
+ "name": "Eme",
+ "pos_x": 33.71875,
+ "pos_y": 26.21875,
+ "pos_z": 4.03125,
+ "stations": "Pausch City,Cabana Hub,Linenger Enterprise,Belyayev Barracks,Lazutkin Settlement"
+ },
+ {
+ "name": "En Kamis",
+ "pos_x": -98.9375,
+ "pos_y": 57.46875,
+ "pos_z": -137.21875,
+ "stations": "Lamarck's Progress,Wiberg Landing,Virchow Port"
+ },
+ {
+ "name": "En Ku Shun",
+ "pos_x": 69.59375,
+ "pos_y": -0.5625,
+ "pos_z": -83.8125,
+ "stations": "Fontenay Works,Fearn Stop"
+ },
+ {
+ "name": "En Kua",
+ "pos_x": -66,
+ "pos_y": 9.84375,
+ "pos_z": -144.8125,
+ "stations": "Selous' Inheritance"
+ },
+ {
+ "name": "En Kungu",
+ "pos_x": -60.09375,
+ "pos_y": 146.34375,
+ "pos_z": 10.53125,
+ "stations": "Silverberg Orbital,Barnes Terminal"
+ },
+ {
+ "name": "En Kunthurs",
+ "pos_x": 16.6875,
+ "pos_y": -85.21875,
+ "pos_z": -99,
+ "stations": "Carlisle Ring,Taylor Dock,Tokubei Keep,Rusch Station,Morrison Terminal"
+ },
+ {
+ "name": "Enawai",
+ "pos_x": 94.375,
+ "pos_y": 67.34375,
+ "pos_z": -83,
+ "stations": "Abe Terminal"
+ },
+ {
+ "name": "Enawar",
+ "pos_x": -63.75,
+ "pos_y": -78.65625,
+ "pos_z": 86.28125,
+ "stations": "Adams Dock"
+ },
+ {
+ "name": "Enaworka",
+ "pos_x": 11.6875,
+ "pos_y": -158.21875,
+ "pos_z": 40.8125,
+ "stations": "Kaiser Dock,Humason City"
+ },
+ {
+ "name": "Enayex",
+ "pos_x": 45.59375,
+ "pos_y": -51.90625,
+ "pos_z": -39.46875,
+ "stations": "Coleman Relay,Watt Port"
+ },
+ {
+ "name": "Enbilulu",
+ "pos_x": 104.375,
+ "pos_y": -2.15625,
+ "pos_z": 1.28125,
+ "stations": "Born Station"
+ },
+ {
+ "name": "Enchimanda",
+ "pos_x": 109.0625,
+ "pos_y": -26.5625,
+ "pos_z": 89.28125,
+ "stations": "Herschel Vision,Roelofs Enterprise"
+ },
+ {
+ "name": "Endin",
+ "pos_x": 128.5,
+ "pos_y": 106.59375,
+ "pos_z": 72.03125,
+ "stations": "Davis Mines,Sheffield Prospect"
+ },
+ {
+ "name": "Endr",
+ "pos_x": 38.90625,
+ "pos_y": 75.9375,
+ "pos_z": -39.03125,
+ "stations": "Mitchell Point"
+ },
+ {
+ "name": "Enek",
+ "pos_x": 37.25,
+ "pos_y": 32.71875,
+ "pos_z": -87.28125,
+ "stations": "Milnor Port,Carr Station,Lewitt Horizons,Chios Depot"
+ },
+ {
+ "name": "Enekpengyi",
+ "pos_x": -24.53125,
+ "pos_y": 59.25,
+ "pos_z": 120.8125,
+ "stations": "Bessemer Hub,Barreiros Beacon"
+ },
+ {
+ "name": "Enet",
+ "pos_x": 88.3125,
+ "pos_y": -41.625,
+ "pos_z": 120,
+ "stations": "Korolyov Orbital,Melia Orbital,Fabricius Port,Baille Hub"
+ },
+ {
+ "name": "Enete",
+ "pos_x": -94.28125,
+ "pos_y": -15.25,
+ "pos_z": -21.5,
+ "stations": "Hamilton Retreat"
+ },
+ {
+ "name": "Enetet",
+ "pos_x": 27.84375,
+ "pos_y": -76.5,
+ "pos_z": -102.6875,
+ "stations": "Meikle Holdings,Grissom Vision,Cook Settlement"
+ },
+ {
+ "name": "Enets",
+ "pos_x": -99.34375,
+ "pos_y": -80.71875,
+ "pos_z": -120,
+ "stations": "Goeppert-Mayer Landing"
+ },
+ {
+ "name": "Enga",
+ "pos_x": 40.71875,
+ "pos_y": 94.8125,
+ "pos_z": -49.3125,
+ "stations": "Babcock Orbital,Vittori Hub,Wafer Observatory"
+ },
+ {
+ "name": "Englar",
+ "pos_x": -26.625,
+ "pos_y": -96.0625,
+ "pos_z": 8.3125,
+ "stations": "Ayerdhal Settlement,Cadamosto Arsenal,Serre Terminal"
+ },
+ {
+ "name": "Enki",
+ "pos_x": -68.0625,
+ "pos_y": 70.78125,
+ "pos_z": -37.78125,
+ "stations": "Bass Port,Ponce de Leon Vision,McAuley Horizons"
+ },
+ {
+ "name": "Ennead",
+ "pos_x": 40.6875,
+ "pos_y": -26.59375,
+ "pos_z": 3.71875,
+ "stations": "Watt Ring,Laplace Port,Gohar Hub,Resnik Station,Blaha Hub,Ivanchenkov Hub,Russell Hub,Andrews Installation,Whitson Depot"
+ },
+ {
+ "name": "Ensoreus",
+ "pos_x": 69.15625,
+ "pos_y": 66.21875,
+ "pos_z": 69.5625,
+ "stations": "Ivanov Station,Slayton Orbital,Phillips Gateway,Bacon Station,Sommerfeld City,Flynn Bastion,Terry Works,Shinn Installation"
+ },
+ {
+ "name": "Enuma",
+ "pos_x": 82.125,
+ "pos_y": -14.125,
+ "pos_z": -76.125,
+ "stations": "Antonelli Colony,Buffett Dock,Pennington Survey"
+ },
+ {
+ "name": "Enumalis",
+ "pos_x": 41.21875,
+ "pos_y": 116.84375,
+ "pos_z": 46.71875,
+ "stations": "Hilmers Port,Babcock Landing"
+ },
+ {
+ "name": "Eochi",
+ "pos_x": 18.25,
+ "pos_y": -250.375,
+ "pos_z": 44.09375,
+ "stations": "Lyulka Colony,Vaucouleurs Mines"
+ },
+ {
+ "name": "Eochumlia",
+ "pos_x": 30.625,
+ "pos_y": -43.21875,
+ "pos_z": 88.78125,
+ "stations": "Tombaugh Installation,Altman Dock,Edison Port"
+ },
+ {
+ "name": "Eol Procul Centauri",
+ "pos_x": -9521.875,
+ "pos_y": -919.40625,
+ "pos_z": 19802.09375,
+ "stations": "Fort Mug"
+ },
+ {
+ "name": "Eol Prou LW-L c8-127",
+ "pos_x": -9528.84375,
+ "pos_y": -913.25,
+ "pos_z": 19809.3125,
+ "stations": "Odin's Crag"
+ },
+ {
+ "name": "Eorasa",
+ "pos_x": -103.5,
+ "pos_y": -98.9375,
+ "pos_z": 66.5,
+ "stations": "Leonov Enterprise,Vasyutin Dock,Bear Beacon"
+ },
+ {
+ "name": "Eos",
+ "pos_x": -51.875,
+ "pos_y": 16.78125,
+ "pos_z": -0.375,
+ "stations": "Bloomfield Orbital,Farrukh Barracks"
+ },
+ {
+ "name": "Eoti",
+ "pos_x": -6.75,
+ "pos_y": -13.09375,
+ "pos_z": 142,
+ "stations": "Morgan Port"
+ },
+ {
+ "name": "Eotienses",
+ "pos_x": 49.5,
+ "pos_y": -104.03125,
+ "pos_z": 6.3125,
+ "stations": "van Royen Survey,Parkinson Dock,Kaufmanis Port,MacCurdy Enterprise,Roe Dock,Adams Market,Peltier Dock,Gray Settlement,Westphal Port,Stein's Folly,Ritchey Port,Delporte Dock,Petlyakov City"
+ },
+ {
+ "name": "Eoto",
+ "pos_x": -101.1875,
+ "pos_y": 44.65625,
+ "pos_z": -86.125,
+ "stations": "Ehrlich Platform,Onizuka Depot"
+ },
+ {
+ "name": "Eototari",
+ "pos_x": -97.96875,
+ "pos_y": -80.21875,
+ "pos_z": -147.34375,
+ "stations": "Taine's Progress,Rocklynne Hub,Jeschke Terminal"
+ },
+ {
+ "name": "Eototo",
+ "pos_x": -118.4375,
+ "pos_y": 18.59375,
+ "pos_z": 100.40625,
+ "stations": "Kurland Port,Smith Enterprise"
+ },
+ {
+ "name": "EP Eridani",
+ "pos_x": 3.84375,
+ "pos_y": -28.53125,
+ "pos_z": -17.65625,
+ "stations": "Godwin Ring,Moore Palace,Virchow Orbital,Tito Terminal,Atiyah Plant"
+ },
+ {
+ "name": "Epoma",
+ "pos_x": 132.0625,
+ "pos_y": -59.125,
+ "pos_z": 52.1875,
+ "stations": "McKean's Progress,Molchanov Orbital,Kirk Port,Trujillo Terminal,Argelander Installation"
+ },
+ {
+ "name": "Epomalinii",
+ "pos_x": -161.34375,
+ "pos_y": 44.625,
+ "pos_z": 1.53125,
+ "stations": "Vizcaino Mines"
+ },
+ {
+ "name": "Epomamo",
+ "pos_x": 67.03125,
+ "pos_y": -44.5,
+ "pos_z": 45.09375,
+ "stations": "Macarthur Terminal,Rustah Prospect"
+ },
+ {
+ "name": "Epomana",
+ "pos_x": 19.40625,
+ "pos_y": 17.53125,
+ "pos_z": 123.3125,
+ "stations": "Cousin Settlement,Onnes Orbital,Leestma Lab"
+ },
+ {
+ "name": "Epomani",
+ "pos_x": -161.71875,
+ "pos_y": -50.15625,
+ "pos_z": -4.5625,
+ "stations": "Carlisle Oasis,Drake Depot,Perga Vision"
+ },
+ {
+ "name": "Epomatz",
+ "pos_x": -48.03125,
+ "pos_y": -137.4375,
+ "pos_z": 101.09375,
+ "stations": "Fuca Enterprise,Binder Holdings,Wilhelm Terminal"
+ },
+ {
+ "name": "Epsilon Andromedae",
+ "pos_x": -118.34375,
+ "pos_y": -89.78125,
+ "pos_z": -69.09375,
+ "stations": "Barbaro Dock,Thompson Colony,Adams Point"
+ },
+ {
+ "name": "Epsilon Ceti",
+ "pos_x": 5.78125,
+ "pos_y": -76.21875,
+ "pos_z": -43.96875,
+ "stations": "Hennen Orbital"
+ },
+ {
+ "name": "Epsilon Cygni",
+ "pos_x": -70.28125,
+ "pos_y": -7.53125,
+ "pos_z": 17.03125,
+ "stations": "Grant Terminal,Rubruck Orbital,Zebrowski Hub"
+ },
+ {
+ "name": "Epsilon Eridani",
+ "pos_x": 1.9375,
+ "pos_y": -7.75,
+ "pos_z": -6.84375,
+ "stations": "Davies Station,Galiano Depot,Betancourt Vision,Fortress Cousens,Darkes High,Kirk Landing,Linaweaver Landing"
+ },
+ {
+ "name": "Epsilon Fornacis",
+ "pos_x": 34.46875,
+ "pos_y": -91.625,
+ "pos_z": -38.03125,
+ "stations": "Houtman Terminal,Gehry Port,Wolszczan Port"
+ },
+ {
+ "name": "Epsilon Hydri",
+ "pos_x": 100,
+ "pos_y": -109.15625,
+ "pos_z": 33.8125,
+ "stations": "Radian V,Torrens Interstellar Trading Outpost,Blaylock Market"
+ },
+ {
+ "name": "Epsilon Indi",
+ "pos_x": 3.125,
+ "pos_y": -8.875,
+ "pos_z": 7.125,
+ "stations": "Mansfield Orbiter,Schneider Relay,London Relay,Flade Enterprise,Perry Depot,King Silo,Krylov Installation,Rowley Settlement,Nikitin Penal colony,Ross Colony"
+ },
+ {
+ "name": "Epsilon Microscopii",
+ "pos_x": -30.6875,
+ "pos_y": -127.53125,
+ "pos_z": 126.5,
+ "stations": "Paez's Progress,Wilhelm Mines,Friesner's Claim"
+ },
+ {
+ "name": "Epsilon Monocerotis",
+ "pos_x": 53.5,
+ "pos_y": -7.40625,
+ "pos_z": -109.71875,
+ "stations": "Makeev Terminal,Sherrington Dock,Stafford Refinery,Rozhdestvensky Point"
+ },
+ {
+ "name": "Epsilon Pavonis",
+ "pos_x": 55.09375,
+ "pos_y": -54.65625,
+ "pos_z": 70.8125,
+ "stations": "Naubakht Orbital"
+ },
+ {
+ "name": "Epsilon Phoenicis",
+ "pos_x": 29.21875,
+ "pos_y": -135.5625,
+ "pos_z": 39.59375,
+ "stations": "R.G.Marett,Schu-Dax Sanctuary,Kaiser Station,Arnold Schwassmann Installation,Krigstein Bastion"
+ },
+ {
+ "name": "Epsilon Scorpii",
+ "pos_x": 11.875,
+ "pos_y": 6.625,
+ "pos_z": 62.21875,
+ "stations": "Gagarin Enterprise,Maxwell Keep,Eilenberg's Pride,Soddy City,Ansari Gateway,Khrunov City,Crown Dock,Bohr Ring,Popovich Hub,Tokarev Hub,Kelly Keep,Kier Lab,Ingstad's Progress"
+ },
+ {
+ "name": "Epsilon Serpentis",
+ "pos_x": -12.34375,
+ "pos_y": 46.25,
+ "pos_z": 51.65625,
+ "stations": "Delbruck Orbital,Anvil Silo,Roosa Vista,Kimbrough Orbital,Shepherd Port,Drexler Enterprise,O'Leary Beacon,Lazarev Beacon"
+ },
+ {
+ "name": "Epsilon-2 Arae",
+ "pos_x": 36.1875,
+ "pos_y": -11.71875,
+ "pos_z": 80.21875,
+ "stations": "Aikin Depot,Hartlib Orbital"
+ },
+ {
+ "name": "EQ Pegasi",
+ "pos_x": -15.4375,
+ "pos_y": -12.75,
+ "pos_z": -2.5625,
+ "stations": "Merbold Ring,Fuchs Base,Zindell Vista,Blaha Enterprise,Napier Hub"
+ },
+ {
+ "name": "Equaesta",
+ "pos_x": -94.40625,
+ "pos_y": -93.46875,
+ "pos_z": -100.46875,
+ "stations": "Lopez de Villalobos' Claim"
+ },
+ {
+ "name": "Equagei",
+ "pos_x": -161.03125,
+ "pos_y": -1.75,
+ "pos_z": -20.59375,
+ "stations": "Leckie City,Bradshaw Station,Archer Colony,Santos Base"
+ },
+ {
+ "name": "Equalkuni",
+ "pos_x": 98.1875,
+ "pos_y": 94.6875,
+ "pos_z": -30.15625,
+ "stations": "Andreas Base,Fossey's Claim,Lefschetz's Claim"
+ },
+ {
+ "name": "ER 8",
+ "pos_x": 37.28125,
+ "pos_y": 12.65625,
+ "pos_z": 27.8125,
+ "stations": "Boswell Dock,Cockrell Prospect"
+ },
+ {
+ "name": "Er Lanci",
+ "pos_x": 100.125,
+ "pos_y": -183.25,
+ "pos_z": 119.53125,
+ "stations": "Phillips Port"
+ },
+ {
+ "name": "Er Long",
+ "pos_x": 122.0625,
+ "pos_y": -131.75,
+ "pos_z": 124.78125,
+ "stations": "Heinkel Landing"
+ },
+ {
+ "name": "Er Longs",
+ "pos_x": 60.4375,
+ "pos_y": -79.34375,
+ "pos_z": -64.34375,
+ "stations": "Hoyle Orbital,He Port,Westerfeld Hub,Meyrink Palace,Dunyach Vision,Sternberg Station,Beebe Settlement"
+ },
+ {
+ "name": "Er Tche",
+ "pos_x": 139.65625,
+ "pos_y": -15.40625,
+ "pos_z": -24.8125,
+ "stations": "Oersted Terminal"
+ },
+ {
+ "name": "Er Tchelis",
+ "pos_x": -22.1875,
+ "pos_y": 2.28125,
+ "pos_z": 158.96875,
+ "stations": "Banks Depot,Skvortsov Prospect"
+ },
+ {
+ "name": "Er Tcher",
+ "pos_x": -83.34375,
+ "pos_y": 139.875,
+ "pos_z": -60.8125,
+ "stations": "Kelly Landing,Drake Estate"
+ },
+ {
+ "name": "Eranin",
+ "pos_x": -22.84375,
+ "pos_y": 36.53125,
+ "pos_z": -1.1875,
+ "stations": "Azeban City,Azeban Orbital,Bordage Works,Eranin 4 Survey"
+ },
+ {
+ "name": "Eravallan",
+ "pos_x": -56.3125,
+ "pos_y": 47.96875,
+ "pos_z": 59.15625,
+ "stations": "Al-Kashi Vision,Shaw Beacon"
+ },
+ {
+ "name": "Eravapa",
+ "pos_x": 77.4375,
+ "pos_y": -60.78125,
+ "pos_z": 68.375,
+ "stations": "Bohr Orbital,Jean Installation,Kandrup Station,Janifer Plant,Brothers Hub"
+ },
+ {
+ "name": "Eravarenth",
+ "pos_x": 66.84375,
+ "pos_y": 21.84375,
+ "pos_z": 27.46875,
+ "stations": "Celsius Terminal,Cooper Landing"
+ },
+ {
+ "name": "Eravate",
+ "pos_x": -42.4375,
+ "pos_y": -3.15625,
+ "pos_z": 59.65625,
+ "stations": "Ackerman Market,Russell Ring,Cleve Hub,McMahon Dock,Tiptree Gateway,Toll Holdings,Sylvester City,Maine Hub"
+ },
+ {
+ "name": "Eravians",
+ "pos_x": 75.28125,
+ "pos_y": 8.53125,
+ "pos_z": -143.5,
+ "stations": "Thuot Gateway,Borlaug Ring,Godwin Orbital,Le Guin Prospect,McMonagle Bastion"
+ },
+ {
+ "name": "Eravins",
+ "pos_x": -32.84375,
+ "pos_y": -253.96875,
+ "pos_z": 27.15625,
+ "stations": "Mobius Mine,Celsius Relay"
+ },
+ {
+ "name": "Erdi",
+ "pos_x": -2.28125,
+ "pos_y": -70.78125,
+ "pos_z": 113.65625,
+ "stations": "Filipchenko Ring,Bresnik Orbital"
+ },
+ {
+ "name": "Erh Lohra",
+ "pos_x": 80.1875,
+ "pos_y": -80.1875,
+ "pos_z": -8.71875,
+ "stations": "Ayton Innes Port,Pilyugin Vision,Ashman Hub,Kaiser Station"
+ },
+ {
+ "name": "Erh Longo",
+ "pos_x": -1.0625,
+ "pos_y": -7.125,
+ "pos_z": 112.125,
+ "stations": "Brongniart City,Virts Station,Shiner Vista"
+ },
+ {
+ "name": "Erio",
+ "pos_x": 30.28125,
+ "pos_y": -93.46875,
+ "pos_z": 88.78125,
+ "stations": "Babakin Hub,Johri Gateway,Spassky Landing,Schaumasse Prospect"
+ },
+ {
+ "name": "Erisagirari",
+ "pos_x": -8.34375,
+ "pos_y": -87.71875,
+ "pos_z": 1.59375,
+ "stations": "Edwards Terminal"
+ },
+ {
+ "name": "Erisha",
+ "pos_x": 125.15625,
+ "pos_y": -131.3125,
+ "pos_z": 78.8125,
+ "stations": "Addams Base"
+ },
+ {
+ "name": "Erishiko",
+ "pos_x": -48.59375,
+ "pos_y": 86.9375,
+ "pos_z": 34.03125,
+ "stations": "Shirley Dock,Tucker Vision,Carpini Keep"
+ },
+ {
+ "name": "Eriusas",
+ "pos_x": 54.53125,
+ "pos_y": 29.8125,
+ "pos_z": 172.5625,
+ "stations": "Pellegrino Platform"
+ },
+ {
+ "name": "Erivara",
+ "pos_x": -17.3125,
+ "pos_y": -75.15625,
+ "pos_z": 121.125,
+ "stations": "Fischer Colony,Oberth Hub,Coulomb Refinery"
+ },
+ {
+ "name": "Erivia",
+ "pos_x": 76.875,
+ "pos_y": -80.59375,
+ "pos_z": 40.0625,
+ "stations": "Cottenot Hub,Norton Survey,Stevenson City"
+ },
+ {
+ "name": "Erivit",
+ "pos_x": 86.53125,
+ "pos_y": 35.5625,
+ "pos_z": -39.34375,
+ "stations": "Garratt Orbital"
+ },
+ {
+ "name": "Erlik",
+ "pos_x": 51.90625,
+ "pos_y": -128.5625,
+ "pos_z": 22.25,
+ "stations": "Reynolds Terminal,Fawcett Penal colony"
+ },
+ {
+ "name": "Erne",
+ "pos_x": -1.0625,
+ "pos_y": -11.8125,
+ "pos_z": -41.375,
+ "stations": "Fisher Orbital,Bohr Port,Soddy Refinery"
+ },
+ {
+ "name": "Ernueken",
+ "pos_x": 26.71875,
+ "pos_y": -41.28125,
+ "pos_z": 87.125,
+ "stations": "Delany Base,Bykovsky Landing"
+ },
+ {
+ "name": "Ernun",
+ "pos_x": 75.6875,
+ "pos_y": 83.15625,
+ "pos_z": -67.3125,
+ "stations": "Holberg Landing,Ibold Base"
+ },
+ {
+ "name": "Ernutef",
+ "pos_x": -46.53125,
+ "pos_y": -93.0625,
+ "pos_z": 1.21875,
+ "stations": "Ross Installation,Sohl Port"
+ },
+ {
+ "name": "Ernutet",
+ "pos_x": -112.75,
+ "pos_y": 74.28125,
+ "pos_z": 25.4375,
+ "stations": "Wiberg Colony,Belyanin Settlement,Wakata Point"
+ },
+ {
+ "name": "Esautama",
+ "pos_x": 57.40625,
+ "pos_y": -43.5625,
+ "pos_z": 86.8125,
+ "stations": "Buckell Enterprise,Dyr Hub,Bugrov Relay"
+ },
+ {
+ "name": "Esautas",
+ "pos_x": 82.375,
+ "pos_y": 104.40625,
+ "pos_z": 60.375,
+ "stations": "Levchenko Settlement,Vishweswarayya Plant,Sheffield Enterprise,Mattingly Asylum"
+ },
+ {
+ "name": "Ese Aibe",
+ "pos_x": 42.59375,
+ "pos_y": 38.46875,
+ "pos_z": -127.4375,
+ "stations": "Baffin Penal colony"
+ },
+ {
+ "name": "Ese Buj",
+ "pos_x": -11.5625,
+ "pos_y": -143.78125,
+ "pos_z": -75.46875,
+ "stations": "Parkinson Orbital,Hickam Port,Swift Terminal,Szentmartony Landing"
+ },
+ {
+ "name": "Ese Eyo",
+ "pos_x": 148.125,
+ "pos_y": -71.4375,
+ "pos_z": 86.4375,
+ "stations": "Curtis Dock,Rayhan al-Biruni Installation"
+ },
+ {
+ "name": "Eseasa",
+ "pos_x": 36.78125,
+ "pos_y": -153.9375,
+ "pos_z": 17.71875,
+ "stations": "Vavrova Ring,Kizawa Station,Giunta Ring,Lange Port,Sabine Ring,Albitzky Station,Allen Base,Nilson Vision,Knight Vista,de Andrade Observatory,Nouvel Relay,Darwin Base"
+ },
+ {
+ "name": "Eshu",
+ "pos_x": 120.78125,
+ "pos_y": -247.1875,
+ "pos_z": -16.4375,
+ "stations": "Shajn Terminal,Celsius Installation"
+ },
+ {
+ "name": "Esien Ming",
+ "pos_x": 32.65625,
+ "pos_y": 69.59375,
+ "pos_z": 59.59375,
+ "stations": "Cortes Dock,Thomas Works,Ibold Point,Cortes Survey,Hardy Camp"
+ },
+ {
+ "name": "Esien Zu",
+ "pos_x": 160.5625,
+ "pos_y": -132.71875,
+ "pos_z": -54.84375,
+ "stations": "Whitford Platform"
+ },
+ {
+ "name": "Eskimori",
+ "pos_x": 47.84375,
+ "pos_y": 12.875,
+ "pos_z": 129.5625,
+ "stations": "Crook Station,Bering Port,Boole Ring,Halsell Keep"
+ },
+ {
+ "name": "Eskit",
+ "pos_x": -153.0625,
+ "pos_y": 6.34375,
+ "pos_z": 50.9375,
+ "stations": "Stuart Mines"
+ },
+ {
+ "name": "Eskite",
+ "pos_x": 16.28125,
+ "pos_y": -138,
+ "pos_z": -47.9375,
+ "stations": "Truman Hub,Paola Port,Sturgeon Vision,Baxter Arsenal"
+ },
+ {
+ "name": "Estae",
+ "pos_x": 17.9375,
+ "pos_y": -62.625,
+ "pos_z": -89.5625,
+ "stations": "Cogswell Dock,Mitchell Colony,Maddox Dock,Ocampo Relay,Butler Vision"
+ },
+ {
+ "name": "Estanatlehi",
+ "pos_x": 169.0625,
+ "pos_y": -167.84375,
+ "pos_z": 96.65625,
+ "stations": "Burnham Survey"
+ },
+ {
+ "name": "Estarna",
+ "pos_x": 148.84375,
+ "pos_y": -194.28125,
+ "pos_z": 106.375,
+ "stations": "Maskelyne Mines"
+ },
+ {
+ "name": "Esubii",
+ "pos_x": 79.1875,
+ "pos_y": -205.21875,
+ "pos_z": 59.40625,
+ "stations": "Lilienthal Point"
+ },
+ {
+ "name": "Esubilanque",
+ "pos_x": 90.65625,
+ "pos_y": -78.5625,
+ "pos_z": -25.5625,
+ "stations": "Eggen Station,Kamov Vision,Bowen Orbital"
+ },
+ {
+ "name": "Esumaramuxa",
+ "pos_x": 83.8125,
+ "pos_y": -84.5,
+ "pos_z": 133.9375,
+ "stations": "Petlyakov's Claim"
+ },
+ {
+ "name": "Esumasci",
+ "pos_x": -117.6875,
+ "pos_y": -120.4375,
+ "pos_z": -8.15625,
+ "stations": "McMahon Gateway,Schouten Vision,Crown Dock,Kinsey Vision"
+ },
+ {
+ "name": "Esumindii",
+ "pos_x": 44.875,
+ "pos_y": 31.5625,
+ "pos_z": 56.40625,
+ "stations": "Nourse City,Brillant Prospect"
+ },
+ {
+ "name": "Esuseku",
+ "pos_x": -107.875,
+ "pos_y": 29.5625,
+ "pos_z": -20.9375,
+ "stations": "Savinykh Orbital,Deluc Port,Savitskaya Hub,Hackworth Dock,Clifford Hub,Wilmore Orbital,Lonchakov Terminal,Cochrane's Pride,Friesner Legacy,Clough Prospect"
+ },
+ {
+ "name": "Esuvit",
+ "pos_x": -13.1875,
+ "pos_y": -1.15625,
+ "pos_z": -92.6875,
+ "stations": "Wilhelm Vision,Payne Settlement,Filter Beacon,Lowry Orbital,Tarelkin Survey"
+ },
+ {
+ "name": "Eta Antliae",
+ "pos_x": 104.84375,
+ "pos_y": 28.25,
+ "pos_z": -2.875,
+ "stations": "Foda Works,Glidden Dock,Froude Barracks"
+ },
+ {
+ "name": "Eta Apodis",
+ "pos_x": 104.78125,
+ "pos_y": -45.0625,
+ "pos_z": 77.8125,
+ "stations": "Swift Oasis,Weiss Relay,Kreutz Landing,Minkowski Colony,Ashman Station"
+ },
+ {
+ "name": "Eta Aquarii",
+ "pos_x": -104.1875,
+ "pos_y": -124.59375,
+ "pos_z": 42.5625,
+ "stations": "Ashton Relay,Lee Dock,He Hub"
+ },
+ {
+ "name": "Eta Cassiopeiae",
+ "pos_x": -16.25,
+ "pos_y": -1.625,
+ "pos_z": -10.5625,
+ "stations": "Angus Manwaring,J.F.Kennedy,Wilcutt Terminal,Watts Oasis,Kessel Silo,Morgue's Mortuary"
+ },
+ {
+ "name": "Eta Cephei",
+ "pos_x": -45.125,
+ "pos_y": 9.375,
+ "pos_z": -6.4375,
+ "stations": "Reilly Plant,Stefanyshyn-Piper's Inheritance"
+ },
+ {
+ "name": "Eta Coronae Borealis",
+ "pos_x": -23.78125,
+ "pos_y": 48.4375,
+ "pos_z": 21.9375,
+ "stations": "Padalka Terminal,Friesner Point,Land Lab,Whitney Point,Franke Horizons,Nagata's Folly"
+ },
+ {
+ "name": "Eta Corvi",
+ "pos_x": 36.71875,
+ "pos_y": 43.03125,
+ "pos_z": 18.78125,
+ "stations": "Williams Hub,Henslow City,Morris' Folly"
+ },
+ {
+ "name": "Eta Crucis",
+ "pos_x": 56.5625,
+ "pos_y": -2.6875,
+ "pos_z": 30.71875,
+ "stations": "Piserchia's Folly,Polyakov Survey,Midgeley Mines"
+ },
+ {
+ "name": "Eta Draconis",
+ "pos_x": -69.53125,
+ "pos_y": 60.28125,
+ "pos_z": -2.9375,
+ "stations": "Alvares Terminal,Swift Terminal,Dietz Orbital,Fisk Port,Fozard Hub,Vinge Port,Pelt Hub,Obruchev Market,Irens Hub"
+ },
+ {
+ "name": "Eta Horologii",
+ "pos_x": 79.1875,
+ "pos_y": -125.71875,
+ "pos_z": 2.34375,
+ "stations": "Johri Escape,Naubakht City,Diamond Depot,Freycinet Observatory"
+ },
+ {
+ "name": "Eta Indi",
+ "pos_x": 13.9375,
+ "pos_y": -49.34375,
+ "pos_z": 59.875,
+ "stations": "Glidden Terminal,Kuo Terminal,Hornby Dock,Zindell's Progress"
+ },
+ {
+ "name": "Eta Leporis",
+ "pos_x": 29.65625,
+ "pos_y": -15,
+ "pos_z": -35.375,
+ "stations": "Moran Station,Cleve Depot,Nachtigal City"
+ },
+ {
+ "name": "Eta Sagittarii",
+ "pos_x": 8.09375,
+ "pos_y": -26.03125,
+ "pos_z": 143.34375,
+ "stations": "Coles Orbital,Kier Enterprise,Francisco de Eliza Dock"
+ },
+ {
+ "name": "Eta Scorpii",
+ "pos_x": 19.34375,
+ "pos_y": -3.6875,
+ "pos_z": 70.78125,
+ "stations": "Hardwick Ring,Fermi Landing"
+ },
+ {
+ "name": "Eta Serpentis",
+ "pos_x": -27.59375,
+ "pos_y": 5.03125,
+ "pos_z": 53.5625,
+ "stations": "Haipeng Port,Ray Dock,Baker Enterprise,Carver Station,Flade Orbital,Crippen Ring,Qurra Ring,Galois Beacon,Cogswell Hub,Fincke Laboratory"
+ },
+ {
+ "name": "Eta Telescopii",
+ "pos_x": 40.75,
+ "pos_y": -70.84375,
+ "pos_z": 134.34375,
+ "stations": "Kibalchich Mines,Hickman Platform,Sekowski's Progress"
+ },
+ {
+ "name": "Eta-1 Pictoris",
+ "pos_x": 65.40625,
+ "pos_y": -51.6875,
+ "pos_z": -16.875,
+ "stations": "Alvares Prospect,Goeschke Station,Hale Orbital,Popov Ring,Bhabha Orbital,Culbertson Ring,Poleshchuk Hub,Kekule Dock,Marshall Hub,Roddenberry Depot,Northrop Orbital"
+ },
+ {
+ "name": "Eta-2 Hydri",
+ "pos_x": 132.6875,
+ "pos_y": -163.9375,
+ "pos_z": 58.03125,
+ "stations": "Tennyson d'Eyncourt Port,Shiras Port,Bloch Enterprise,Bordage Orbital"
+ },
+ {
+ "name": "Etain",
+ "pos_x": 12.5,
+ "pos_y": 35.03125,
+ "pos_z": -37.1875,
+ "stations": "Stafford Vision,Levi-Montalcini City,Vian Landing,Berliner Refinery"
+ },
+ {
+ "name": "Etamin",
+ "pos_x": -132.46875,
+ "pos_y": 74.875,
+ "pos_z": 25.53125,
+ "stations": "Smith Port,Fincke Mines,Maybury's Claim"
+ },
+ {
+ "name": "Ethen",
+ "pos_x": 131.78125,
+ "pos_y": 2.71875,
+ "pos_z": -18.5625,
+ "stations": "Cochrane Survey,Reamy Dock,Forbes Beacon,Chasles Dock,Euthymenes Dock"
+ },
+ {
+ "name": "Ethere",
+ "pos_x": -92.5625,
+ "pos_y": -44.9375,
+ "pos_z": 27.8125,
+ "stations": "Meade Plant,Eyharts Platform"
+ },
+ {
+ "name": "Ethet",
+ "pos_x": 43.90625,
+ "pos_y": 27.1875,
+ "pos_z": 100.6875,
+ "stations": "Morgan Installation"
+ },
+ {
+ "name": "Ethgreze",
+ "pos_x": -30.03125,
+ "pos_y": 72.34375,
+ "pos_z": -23.8125,
+ "stations": "Bloch Station,Quimper Penal colony"
+ },
+ {
+ "name": "Ethli",
+ "pos_x": 3.1875,
+ "pos_y": -4.65625,
+ "pos_z": -83.21875,
+ "stations": "Gurragchaa Station,Halsell Station,Stairs Bastion"
+ },
+ {
+ "name": "Ethwain",
+ "pos_x": -24.46875,
+ "pos_y": 131.1875,
+ "pos_z": 36.28125,
+ "stations": "Shalatula Gateway"
+ },
+ {
+ "name": "Etmanei",
+ "pos_x": 85.25,
+ "pos_y": -100.84375,
+ "pos_z": -74.9375,
+ "stations": "Crumey Horizons,Clerk Port,Ellis Station"
+ },
+ {
+ "name": "Etmang",
+ "pos_x": -120.125,
+ "pos_y": -91.625,
+ "pos_z": 7.46875,
+ "stations": "Onnes Installation,Evans Colony,Maire Beacon"
+ },
+ {
+ "name": "Etz'nama",
+ "pos_x": 155.25,
+ "pos_y": -14.28125,
+ "pos_z": -61.84375,
+ "stations": "Carey Beacon"
+ },
+ {
+ "name": "Etz'nambe",
+ "pos_x": 112.6875,
+ "pos_y": -158.9375,
+ "pos_z": -51.59375,
+ "stations": "Lippisch Platform,Zhukovsky Sanctuary,Kidd Reach"
+ },
+ {
+ "name": "Etznab Yu",
+ "pos_x": 60.875,
+ "pos_y": -202.375,
+ "pos_z": 121.1875,
+ "stations": "Leuschner Port"
+ },
+ {
+ "name": "Etznabal",
+ "pos_x": 75.125,
+ "pos_y": -219.375,
+ "pos_z": -59.375,
+ "stations": "Dowie Platform,Whelan Gateway,Goldberg Gateway"
+ },
+ {
+ "name": "Etznabi",
+ "pos_x": 64,
+ "pos_y": -159.625,
+ "pos_z": -67.25,
+ "stations": "Mayor Dock,Espenak Platform,de Seversky Port,van Houten Depot"
+ },
+ {
+ "name": "Etznabozo",
+ "pos_x": 75.71875,
+ "pos_y": 114.09375,
+ "pos_z": 55.03125,
+ "stations": "Schilling Camp,Milnor Arena"
+ },
+ {
+ "name": "Euboa",
+ "pos_x": -7.53125,
+ "pos_y": -24.15625,
+ "pos_z": 41.5,
+ "stations": "Kadenyuk Hub,Foreman Orbital,Gauss Port,Ledyard Holdings"
+ },
+ {
+ "name": "Eubuju",
+ "pos_x": -69.46875,
+ "pos_y": -97.1875,
+ "pos_z": 152.625,
+ "stations": "Barjavel Dock,Morris Port,Kozlov's Inheritance"
+ },
+ {
+ "name": "Euburiates",
+ "pos_x": -145.625,
+ "pos_y": -17.15625,
+ "pos_z": -2.875,
+ "stations": "Manning Hub,Slade Prospect,Ross Ring,Resnick Hub,Yourkevitch Keep"
+ },
+ {
+ "name": "Eulexia",
+ "pos_x": -3.5,
+ "pos_y": 47.15625,
+ "pos_z": 37.90625,
+ "stations": "Robinson Orbital,Noon Base,Mad J Wagar's,Beekman's Progress"
+ },
+ {
+ "name": "Euripus",
+ "pos_x": 3.8125,
+ "pos_y": 16.03125,
+ "pos_z": -45.75,
+ "stations": "Blalock Horizons,Ray Depot"
+ },
+ {
+ "name": "Euryale",
+ "pos_x": 35.375,
+ "pos_y": -68.96875,
+ "pos_z": 24.8125,
+ "stations": "EG Main HQ,Taylor Barracks,Popovich Station,Gantt Enterprise"
+ },
+ {
+ "name": "Eurybia",
+ "pos_x": 51.40625,
+ "pos_y": -54.40625,
+ "pos_z": -30.5,
+ "stations": "Demolition Unlimited,Awyra Flirble,Chris & Silvia's Paradise Hideout,Michell Depot,Gill Point"
+ },
+ {
+ "name": "Evejitaense",
+ "pos_x": -66.25,
+ "pos_y": 52.25,
+ "pos_z": -17.78125,
+ "stations": "Frimout Base,Champlain Base,Otto Outpost"
+ },
+ {
+ "name": "Evejitaka",
+ "pos_x": -18.8125,
+ "pos_y": -63.46875,
+ "pos_z": 57.0625,
+ "stations": "Hume Hub,Bursch Station,Henize Station,Love Ring,Martinez Terminal"
+ },
+ {
+ "name": "Evena",
+ "pos_x": 9.34375,
+ "pos_y": -2.4375,
+ "pos_z": 96.03125,
+ "stations": "Frobisher Settlement,Jemison Ring,Shaver Terminal,Whitworth Ring,Bursch Hub"
+ },
+ {
+ "name": "Evenks",
+ "pos_x": -37.5,
+ "pos_y": 19.65625,
+ "pos_z": -79.34375,
+ "stations": "Buckland Works"
+ },
+ {
+ "name": "Evenses",
+ "pos_x": -133.875,
+ "pos_y": 54.03125,
+ "pos_z": -80.5625,
+ "stations": "Sawyer Dock,Wisniewski-Snerg Orbital,McHugh Terminal,Alexander City"
+ },
+ {
+ "name": "Eventiensi",
+ "pos_x": 89.5,
+ "pos_y": 17.4375,
+ "pos_z": -135.03125,
+ "stations": "Barbaro Dock,Simmons Dock"
+ },
+ {
+ "name": "Eventina",
+ "pos_x": -106.09375,
+ "pos_y": -97.9375,
+ "pos_z": 101.90625,
+ "stations": "Ryazanski Outpost"
+ },
+ {
+ "name": "Evergreen",
+ "pos_x": -86.6875,
+ "pos_y": 25.34375,
+ "pos_z": -3.3125,
+ "stations": "Kazantsev Ring,Forsskal Hub,Kandel Legacy,McCaffrey Enterprise"
+ },
+ {
+ "name": "Evnici",
+ "pos_x": -99.28125,
+ "pos_y": -120.25,
+ "pos_z": -71.625,
+ "stations": "Cousin Ring,Pelliot Gateway,Klein Terminal,Auer Depot,Cartan Base"
+ },
+ {
+ "name": "Evnicoano",
+ "pos_x": 93.15625,
+ "pos_y": 8.15625,
+ "pos_z": -82.1875,
+ "stations": "Crick Prospect,Ings Mine,O'Donnell Mine"
+ },
+ {
+ "name": "Evnike",
+ "pos_x": -146.46875,
+ "pos_y": 48.59375,
+ "pos_z": -19.125,
+ "stations": "Burstein Port,Ings Station,Samos Terminal"
+ },
+ {
+ "name": "Evnis",
+ "pos_x": 64.75,
+ "pos_y": 71.21875,
+ "pos_z": -163.28125,
+ "stations": "Moon Beacon,Nesvadba Platform,Darnielle Prospect"
+ },
+ {
+ "name": "Evnisei",
+ "pos_x": 96.59375,
+ "pos_y": -163.75,
+ "pos_z": -40.21875,
+ "stations": "Plaskett Terminal,Yamamoto Orbital,Sweet Enterprise"
+ },
+ {
+ "name": "Ewah",
+ "pos_x": -129.0625,
+ "pos_y": -12.84375,
+ "pos_z": -18,
+ "stations": "Newton City,Smith Port,Viktorenko City,Noether Silo,Chwedyk Works"
+ },
+ {
+ "name": "Ewahillke",
+ "pos_x": -114.125,
+ "pos_y": -43.875,
+ "pos_z": -53.28125,
+ "stations": "Vinci Survey,MacLean's Inheritance"
+ },
+ {
+ "name": "Ewahu",
+ "pos_x": 160.15625,
+ "pos_y": -157.25,
+ "pos_z": 78.28125,
+ "stations": "Samos Landing,Wasden Station,Mizuno Beacon"
+ },
+ {
+ "name": "Ewambutsu",
+ "pos_x": 83.28125,
+ "pos_y": -165.65625,
+ "pos_z": 23.4375,
+ "stations": "Mrkos Installation,Rees Hub,Tenn Installation,Scotti Vision"
+ },
+ {
+ "name": "Ewe",
+ "pos_x": -108.875,
+ "pos_y": 9.8125,
+ "pos_z": 43.0625,
+ "stations": "Bennett Landing"
+ },
+ {
+ "name": "Ewenet",
+ "pos_x": 111.65625,
+ "pos_y": 128.96875,
+ "pos_z": 54.65625,
+ "stations": "Young Prospect,Geston Colony"
+ },
+ {
+ "name": "Ewenks",
+ "pos_x": 143.6875,
+ "pos_y": 44.59375,
+ "pos_z": -20.5625,
+ "stations": "Kepler Prospect,Davis Platform"
+ },
+ {
+ "name": "Exbeur",
+ "pos_x": -35.15625,
+ "pos_y": 27.84375,
+ "pos_z": -33.65625,
+ "stations": "Engle Hub,Lyakhov Horizons,Haxel Enterprise,Bush High,Wakata Hub,Guidoni's Pride,McNair Vision"
+ },
+ {
+ "name": "Excessis",
+ "pos_x": 25.84375,
+ "pos_y": -30.46875,
+ "pos_z": -83.46875,
+ "stations": "Fiennes Enterprise"
+ },
+ {
+ "name": "Exigus",
+ "pos_x": -2.625,
+ "pos_y": 38.3125,
+ "pos_z": -84.125,
+ "stations": "Slackagers' Hangout,Maudslay Depot"
+ },
+ {
+ "name": "Eximo",
+ "pos_x": 60.28125,
+ "pos_y": -15.3125,
+ "pos_z": -68.34375,
+ "stations": "Barentsz Vision,Shinn Landing,Foden Plant"
+ },
+ {
+ "name": "Exioce",
+ "pos_x": 78.5,
+ "pos_y": -100.25,
+ "pos_z": 16.78125,
+ "stations": "Miller Terminal,Sabine Installation,Fort Lawrence,Macmillan Depot,Reinmuth Landing"
+ },
+ {
+ "name": "Exphiay",
+ "pos_x": 283.84375,
+ "pos_y": -128.25,
+ "pos_z": -38.9375,
+ "stations": "James K Winston,Worlidge Hub,Polansky Legacy"
+ },
+ {
+ "name": "EZ Aquarii",
+ "pos_x": -4.4375,
+ "pos_y": -9.3125,
+ "pos_z": 4.09375,
+ "stations": "Magnus Gateway,Sanger Settlement"
+ },
+ {
+ "name": "Ezerishapa",
+ "pos_x": -99.46875,
+ "pos_y": 0.9375,
+ "pos_z": 82.0625,
+ "stations": "Nespoli Ring"
+ },
+ {
+ "name": "Ezerno",
+ "pos_x": -152.96875,
+ "pos_y": 38.25,
+ "pos_z": 37.15625,
+ "stations": "Wilcutt Orbital,Fulton Relay,Thompson Terminal,Morgan Lab"
+ },
+ {
+ "name": "Facece",
+ "pos_x": 64.28125,
+ "pos_y": -111.4375,
+ "pos_z": 25.1875,
+ "stations": "Fortress York,Simpson Depot,Waldrop Beacon,Secchi Landing,Peters Base,Florine Holdings,VanderMeer Installation,Patrick Depot,Kelly Works,Penrose Port,Arrhenius Hub"
+ },
+ {
+ "name": "Fafnisei",
+ "pos_x": 146.375,
+ "pos_y": -114.875,
+ "pos_z": -46.28125,
+ "stations": "Pennington Beacon,Hay Platform,Whelan Port,Waldrop Base"
+ },
+ {
+ "name": "Falendal",
+ "pos_x": 132.28125,
+ "pos_y": -127,
+ "pos_z": 76,
+ "stations": "Kizawa Vision,Hedin Enterprise,Stiles Gateway,Wenzel Hub"
+ },
+ {
+ "name": "Fali",
+ "pos_x": -130.59375,
+ "pos_y": 43.09375,
+ "pos_z": -41.03125,
+ "stations": "Heaviside Prospect"
+ },
+ {
+ "name": "Falisci",
+ "pos_x": 61.375,
+ "pos_y": -91.0625,
+ "pos_z": -14.8125,
+ "stations": "Moffat Settlement,Madsen Colony,Phillips Landing"
+ },
+ {
+ "name": "Falitabozo",
+ "pos_x": -117.46875,
+ "pos_y": -97.125,
+ "pos_z": 87.875,
+ "stations": "Mach Port,Springer Hub,Volterra Penal colony"
+ },
+ {
+ "name": "Fall",
+ "pos_x": -68.75,
+ "pos_y": 63.1875,
+ "pos_z": 37.4375,
+ "stations": "Babcock Relay"
+ },
+ {
+ "name": "Fan Cechehe",
+ "pos_x": -89.34375,
+ "pos_y": -108.9375,
+ "pos_z": 51.84375,
+ "stations": "Hermite Dock"
+ },
+ {
+ "name": "Fan Chaguti",
+ "pos_x": 115.125,
+ "pos_y": -222.15625,
+ "pos_z": -42.625,
+ "stations": "Auzout Settlement,Tsiolkovsky Terminal"
+ },
+ {
+ "name": "Fan Chau",
+ "pos_x": 66.28125,
+ "pos_y": 18.375,
+ "pos_z": 96.21875,
+ "stations": "Cayley's Progress,Pennington Dock"
+ },
+ {
+ "name": "Fan Gong",
+ "pos_x": -84.65625,
+ "pos_y": -100.46875,
+ "pos_z": 80.59375,
+ "stations": "Brash Settlement"
+ },
+ {
+ "name": "Fan Kui",
+ "pos_x": -29.375,
+ "pos_y": 153.125,
+ "pos_z": -77.96875,
+ "stations": "Ferguson Beacon,Cortes Dock,Pond Ring,Frobisher Settlement"
+ },
+ {
+ "name": "Fan Naom",
+ "pos_x": -136.3125,
+ "pos_y": 107.53125,
+ "pos_z": 4.0625,
+ "stations": "Quimby Settlement"
+ },
+ {
+ "name": "Fan Wen",
+ "pos_x": 186.53125,
+ "pos_y": -138.28125,
+ "pos_z": 43.53125,
+ "stations": "O'Donnell Plant,Banneker Colony,Ptack Reformatory"
+ },
+ {
+ "name": "Fan Yin",
+ "pos_x": 71,
+ "pos_y": 32.125,
+ "pos_z": -53.03125,
+ "stations": "Simpson Ring,Soddy Station,Joule Hub,Dorsey Landing"
+ },
+ {
+ "name": "Fandh",
+ "pos_x": -15.4375,
+ "pos_y": -179.9375,
+ "pos_z": 8.15625,
+ "stations": "Curtiss Mines,Wilhelm Klinkerfues Landing,Hodgson Relay"
+ },
+ {
+ "name": "Fandi",
+ "pos_x": 156.09375,
+ "pos_y": -15.125,
+ "pos_z": 65.78125,
+ "stations": "Shaw Port,Weil Barracks"
+ },
+ {
+ "name": "Fang",
+ "pos_x": 147.71875,
+ "pos_y": -218,
+ "pos_z": 21.5,
+ "stations": "Tange Holdings,Boyajian Terminal,Morris Depot,Frazetta Terminal"
+ },
+ {
+ "name": "Far Tauri",
+ "pos_x": -9510.65625,
+ "pos_y": -915,
+ "pos_z": 19793.34375,
+ "stations": "Chaydar Correctional"
+ },
+ {
+ "name": "Farack",
+ "pos_x": -18.90625,
+ "pos_y": 79.40625,
+ "pos_z": -8.9375,
+ "stations": "Regiomontanus' Pride,Mitra Estate"
+ },
+ {
+ "name": "Faroa",
+ "pos_x": 102.09375,
+ "pos_y": -157.5625,
+ "pos_z": 140.5625,
+ "stations": "Carroll Prospect,Wilson Settlement"
+ },
+ {
+ "name": "Faroahy",
+ "pos_x": -39.28125,
+ "pos_y": -98.8125,
+ "pos_z": 68.59375,
+ "stations": "Fraley Horizons,Lichtenberg City,Makarov Landing,de Andrade Settlement"
+ },
+ {
+ "name": "Faroo",
+ "pos_x": -66.65625,
+ "pos_y": -124.375,
+ "pos_z": 78.625,
+ "stations": "Brunner Silo,Zubrin Terminal"
+ },
+ {
+ "name": "Faroras",
+ "pos_x": 94.4375,
+ "pos_y": -93.03125,
+ "pos_z": 81.71875,
+ "stations": "Hume Prison,Parkinson Works"
+ },
+ {
+ "name": "Faros",
+ "pos_x": 148.5,
+ "pos_y": -158.09375,
+ "pos_z": 110.46875,
+ "stations": "Brundage Silo,Whitcomb Depot"
+ },
+ {
+ "name": "Farowalan",
+ "pos_x": -4.9375,
+ "pos_y": -65.78125,
+ "pos_z": -98.5625,
+ "stations": "Bamford City,Longyear Arsenal"
+ },
+ {
+ "name": "Farwell",
+ "pos_x": -9521.40625,
+ "pos_y": -888.28125,
+ "pos_z": 19845.65625,
+ "stations": "Phoenix Industries"
+ },
+ {
+ "name": "Fasti",
+ "pos_x": 36.71875,
+ "pos_y": 20.1875,
+ "pos_z": -22.3125,
+ "stations": "Gaffney Installation"
+ },
+ {
+ "name": "Fates",
+ "pos_x": -54.5625,
+ "pos_y": 20.75,
+ "pos_z": -62.15625,
+ "stations": "Abasheli Camp,Lyakhov Dock"
+ },
+ {
+ "name": "FAUST 2688",
+ "pos_x": 62.5,
+ "pos_y": 51.4375,
+ "pos_z": -11.46875,
+ "stations": "Vinge Installation,Lousma Orbital"
+ },
+ {
+ "name": "FAUST 3566",
+ "pos_x": 7.125,
+ "pos_y": 109.90625,
+ "pos_z": 7.65625,
+ "stations": "Murphy Gateway,Perry Dock,Collins Dock,Smith Station,Wagner Terminal,Bentham Dock,Pierres Enterprise,Robinson Settlement,Abe Hub,Doyle Base"
+ },
+ {
+ "name": "FAUST 68",
+ "pos_x": 72.34375,
+ "pos_y": -89.90625,
+ "pos_z": 46.59375,
+ "stations": "Slipher Hub,Dominique Prospect,Moon Installation"
+ },
+ {
+ "name": "Fawaol",
+ "pos_x": 65.5625,
+ "pos_y": -118.15625,
+ "pos_z": -10.09375,
+ "stations": "Sutter Hub,Korolev Orbital,Sy Station,Gottlob Frege Coliseum,Morris Settlement,Brom Vision"
+ },
+ {
+ "name": "Fedmich",
+ "pos_x": 23.875,
+ "pos_y": 75.1875,
+ "pos_z": 35.75,
+ "stations": "Roberts Port,Blish Beacon,Ron Hubbard Dock,Effinger Port,Narbeth Depot"
+ },
+ {
+ "name": "Fehu",
+ "pos_x": 46.375,
+ "pos_y": -448.6875,
+ "pos_z": -127.125,
+ "stations": "Frigschneck's Resort,Thompson-Keen Asylum,Lambert Terminal,Aikin's Inheritance"
+ },
+ {
+ "name": "Fei Lin",
+ "pos_x": 21.375,
+ "pos_y": -208.125,
+ "pos_z": -22,
+ "stations": "Benford Survey,Stone Colony,Tousey Mines,Clark Point"
+ },
+ {
+ "name": "Fei Zu",
+ "pos_x": 57.15625,
+ "pos_y": 36.25,
+ "pos_z": 135.3125,
+ "stations": "Gilliland Station,Lasswitz Vision,Stein Penal colony"
+ },
+ {
+ "name": "Feige 22",
+ "pos_x": -16,
+ "pos_y": -65.8125,
+ "pos_z": -54.53125,
+ "stations": "Plexico Point,Buckland Gateway,Atkov Settlement,Allen Gateway,Lovelace Terminal"
+ },
+ {
+ "name": "Feldr",
+ "pos_x": -57.625,
+ "pos_y": 14.8125,
+ "pos_z": 73.0625,
+ "stations": "Musabayev Station,McDaniel Barracks,Ellison Laboratory"
+ },
+ {
+ "name": "Felkan",
+ "pos_x": -34.5,
+ "pos_y": -62.90625,
+ "pos_z": -42.9375,
+ "stations": "Jack's Town,Norgay Arsenal,Oluwafemi Orbital,Pudwill Gorie Orbital,Doctorow Camp"
+ },
+ {
+ "name": "Feng Du",
+ "pos_x": -39.9375,
+ "pos_y": -153.90625,
+ "pos_z": 72.15625,
+ "stations": "Niijima Mines,Arfstrom Arena"
+ },
+ {
+ "name": "Feng Tanga",
+ "pos_x": 5.75,
+ "pos_y": -113.125,
+ "pos_z": -67.3125,
+ "stations": "Weaver Port,Cook Landing,Kelly Port,Daqing Port,Chernykh Terminal"
+ },
+ {
+ "name": "Feng Tu",
+ "pos_x": -114.34375,
+ "pos_y": 80.5625,
+ "pos_z": 101.4375,
+ "stations": "Hermaszewski Gateway,Garay Orbital,Illy Orbital,Baker Bastion,Glenn Settlement"
+ },
+ {
+ "name": "Fengai",
+ "pos_x": -108.6875,
+ "pos_y": -96.0625,
+ "pos_z": 24.625,
+ "stations": "Poincare Prospect,Tem Orbital"
+ },
+ {
+ "name": "Fengalufon",
+ "pos_x": 106.8125,
+ "pos_y": 4.875,
+ "pos_z": 48.53125,
+ "stations": "Mackenzie Point,Vinge's Pride,Nansen Colony"
+ },
+ {
+ "name": "Fenghus",
+ "pos_x": -126.5,
+ "pos_y": -98.59375,
+ "pos_z": -32.125,
+ "stations": "Manning Port,Koontz Gateway"
+ },
+ {
+ "name": "Fengiri",
+ "pos_x": -98.9375,
+ "pos_y": -16.53125,
+ "pos_z": 75.0625,
+ "stations": "Mille Gateway,Clairaut Dock,Crown Settlement"
+ },
+ {
+ "name": "Fengita",
+ "pos_x": -108.53125,
+ "pos_y": 75.625,
+ "pos_z": -16,
+ "stations": "Clough Barracks,Lundwall Hub,Rangarajan Stop"
+ },
+ {
+ "name": "Fengol",
+ "pos_x": 65.65625,
+ "pos_y": -155.625,
+ "pos_z": 90.5,
+ "stations": "Ikeya Settlement,Wenzel Dock"
+ },
+ {
+ "name": "Fengthi",
+ "pos_x": 80.28125,
+ "pos_y": -178.53125,
+ "pos_z": 30.15625,
+ "stations": "Gurevich Vision"
+ },
+ {
+ "name": "Fenricha",
+ "pos_x": -105.15625,
+ "pos_y": -78.375,
+ "pos_z": 12.3125,
+ "stations": "Bailey Vista,Pinzon Stop"
+ },
+ {
+ "name": "Fenrichua",
+ "pos_x": -39.3125,
+ "pos_y": -95.15625,
+ "pos_z": -88.875,
+ "stations": "Aldrin City,Womack Silo,Lindbohm Installation"
+ },
+ {
+ "name": "Fenrisastya",
+ "pos_x": -121.125,
+ "pos_y": -82.375,
+ "pos_z": 49.28125,
+ "stations": "Tepper's Progress"
+ },
+ {
+ "name": "Fer Doira",
+ "pos_x": 83.84375,
+ "pos_y": -8.53125,
+ "pos_z": 106.9375,
+ "stations": "Bailey Dock,Gelfand Vision,Galindo Port,Nylund Arsenal,Dall Station,Ferguson Dock,Minkowski's Progress,Dunyach Port,Barth Escape"
+ },
+ {
+ "name": "Ferenchia",
+ "pos_x": -12.8125,
+ "pos_y": -90.0625,
+ "pos_z": 53.5625,
+ "stations": "Melvin Mine,Edgeworth Survey,Nikolayev Installation"
+ },
+ {
+ "name": "Fermer",
+ "pos_x": -83.875,
+ "pos_y": -18.6875,
+ "pos_z": 18.3125,
+ "stations": "Weber Terminal,Alas Relay"
+ },
+ {
+ "name": "Fetill",
+ "pos_x": 81.625,
+ "pos_y": 49.09375,
+ "pos_z": -7.40625,
+ "stations": "Huygens City,Watson Orbital,Hopkins Settlement,Norman Silo"
+ },
+ {
+ "name": "Feuma",
+ "pos_x": 22.875,
+ "pos_y": 78.625,
+ "pos_z": 23.59375,
+ "stations": "Frobac's Folly,Paulo da Gama Survey"
+ },
+ {
+ "name": "FF Andromedae",
+ "pos_x": -52.6875,
+ "pos_y": -31.6875,
+ "pos_z": -31.59375,
+ "stations": "Efremov Orbital,Murphy Orbital,Gardner Port,Fancher Beacon,Isherwood Works,Weil Silo"
+ },
+ {
+ "name": "Fiden",
+ "pos_x": -57.5,
+ "pos_y": -74.75,
+ "pos_z": -109.125,
+ "stations": "Bradbury Gateway,Panshin Ring,Lynn Hub,Shirley City,Frobenius Base,Butcher Station,Mouhot Gateway"
+ },
+ {
+ "name": "Fidena",
+ "pos_x": 55.96875,
+ "pos_y": -75.78125,
+ "pos_z": -30.84375,
+ "stations": "Schottky Terminal"
+ },
+ {
+ "name": "Fidenef",
+ "pos_x": 38.03125,
+ "pos_y": -24.1875,
+ "pos_z": -23.5625,
+ "stations": "Hyecho Colony,Roddenberry Landing"
+ },
+ {
+ "name": "Fidense",
+ "pos_x": -27.90625,
+ "pos_y": 62.34375,
+ "pos_z": -113.40625,
+ "stations": "Rennie Port,Morey Retreat"
+ },
+ {
+ "name": "Fidenses",
+ "pos_x": 42.4375,
+ "pos_y": -213.53125,
+ "pos_z": 76.5,
+ "stations": "Kulin Landing,Gurevich Vision,Dhawan Installation,Guth Station"
+ },
+ {
+ "name": "Fimbulwinter",
+ "pos_x": -21.125,
+ "pos_y": -8.6875,
+ "pos_z": -100.6875,
+ "stations": "Budrys Laboratory,Engle Terminal,Shatalov Landing"
+ },
+ {
+ "name": "Fincien",
+ "pos_x": -89.25,
+ "pos_y": -25.84375,
+ "pos_z": 82.65625,
+ "stations": "Aldrin Market,Besonders Prospect,Junlong Orbital,Grechko Terminal,Ferguson Survey,Doi Enterprise,Kononenko Orbital,Eyharts Enterprise,Carey Installation,Braun Port,Linenger Port,Nesvadba Landing,Halley Hub,Speke Holdings"
+ },
+ {
+ "name": "Findalibila",
+ "pos_x": 39.5,
+ "pos_y": -44.0625,
+ "pos_z": -30.28125,
+ "stations": "Zajdel Holdings,Grassmann Station,Tasman Dock"
+ },
+ {
+ "name": "Findbhair",
+ "pos_x": 15.59375,
+ "pos_y": -2.90625,
+ "pos_z": -120.8125,
+ "stations": "Yu Point,Kennedy Platform"
+ },
+ {
+ "name": "Findja",
+ "pos_x": 37.625,
+ "pos_y": -81.78125,
+ "pos_z": 45.71875,
+ "stations": "Gaensler Station,Seyfert Installation,Horrocks Terminal"
+ },
+ {
+ "name": "Finepatis",
+ "pos_x": -76.84375,
+ "pos_y": -69.03125,
+ "pos_z": 136.875,
+ "stations": "Utley Installation,Davidson Platform,Blair Depot"
+ },
+ {
+ "name": "Fintamans",
+ "pos_x": 164.78125,
+ "pos_y": -21.96875,
+ "pos_z": 15.34375,
+ "stations": "Walker Orbital,Scalzi Installation,Sekelj Ring,Auld Depot,Bates' Progress,Forward Park"
+ },
+ {
+ "name": "Fintamkina",
+ "pos_x": 6.625,
+ "pos_y": -40.625,
+ "pos_z": 130.6875,
+ "stations": "Gordon Arsenal,Shukor Vision"
+ },
+ {
+ "name": "Fintan",
+ "pos_x": 13.5625,
+ "pos_y": -153.625,
+ "pos_z": -97.15625,
+ "stations": "Apianus Colony,Polikarpov Prospect,Kowal Terminal"
+ },
+ {
+ "name": "Finteno",
+ "pos_x": -106.375,
+ "pos_y": -16.21875,
+ "pos_z": 4.9375,
+ "stations": "Kolmogorov Station,Lewis Base"
+ },
+ {
+ "name": "Finti",
+ "pos_x": 68.15625,
+ "pos_y": -38.4375,
+ "pos_z": 47.71875,
+ "stations": "Boucher Freeport,Ingstad Point"
+ },
+ {
+ "name": "Fintia",
+ "pos_x": 131.28125,
+ "pos_y": -121.5625,
+ "pos_z": 29.21875,
+ "stations": "Ohain Platform,Alcock Relay,Giclas Mines"
+ },
+ {
+ "name": "Fionn",
+ "pos_x": 32.5,
+ "pos_y": 9.3125,
+ "pos_z": -22.75,
+ "stations": "Currie Enterprise,Burnell Terminal,Kondakova Ring"
+ },
+ {
+ "name": "Firbar",
+ "pos_x": 2.53125,
+ "pos_y": -141.3125,
+ "pos_z": 42.375,
+ "stations": "Asclepi Landing,Neumann Orbital"
+ },
+ {
+ "name": "Firboleche",
+ "pos_x": 143.3125,
+ "pos_y": 38.40625,
+ "pos_z": 11.65625,
+ "stations": "Reightler Terminal,Metcalf Camp"
+ },
+ {
+ "name": "Firbon",
+ "pos_x": 66.71875,
+ "pos_y": 142.46875,
+ "pos_z": -30.84375,
+ "stations": "Ferguson Platform,Dann Base,Vinci Works"
+ },
+ {
+ "name": "Firdaus",
+ "pos_x": 62.96875,
+ "pos_y": -89.5625,
+ "pos_z": 35.625,
+ "stations": "Bernoulli Hangar,Robert Aitken Orbital,Lynn Terminal"
+ },
+ {
+ "name": "Fire Fade",
+ "pos_x": 130.8125,
+ "pos_y": -164.09375,
+ "pos_z": 98.5625,
+ "stations": "Tsiolkovsky Ring,Milne Vision,Siddha's Progress,Pearse Station"
+ },
+ {
+ "name": "Fire Flasa",
+ "pos_x": -106.03125,
+ "pos_y": -20.3125,
+ "pos_z": 107.28125,
+ "stations": "Alexandria Settlement,Vela Reach"
+ },
+ {
+ "name": "Firen",
+ "pos_x": -50,
+ "pos_y": -51.9375,
+ "pos_z": 82.625,
+ "stations": "Kronecker Dock,Kingsbury Vision,Reightler Terminal,Effinger Hub,McKee Terminal,Hamilton Dock,Vardeman Survey"
+ },
+ {
+ "name": "Firenses",
+ "pos_x": -88.46875,
+ "pos_y": -63.53125,
+ "pos_z": 31.1875,
+ "stations": "Roberts Gateway,Hill Installation,Quimby Ring"
+ },
+ {
+ "name": "Firey",
+ "pos_x": 58.15625,
+ "pos_y": -37.78125,
+ "pos_z": 105.78125,
+ "stations": "Lem Dock,Elder City"
+ },
+ {
+ "name": "Fjorgyn",
+ "pos_x": 38.375,
+ "pos_y": -43.71875,
+ "pos_z": 42.125,
+ "stations": "Harper Plant,Stevens Base,Humphreys Orbital"
+ },
+ {
+ "name": "FK Aquarii",
+ "pos_x": -9,
+ "pos_y": -24.46875,
+ "pos_z": 11.1875,
+ "stations": "Hurley City,Bowersox Station,Anders Hub,Serrao Hub,Butz Installation"
+ },
+ {
+ "name": "FK5 2550",
+ "pos_x": 17.1875,
+ "pos_y": 22.65625,
+ "pos_z": -75.21875,
+ "stations": "Moorcock Barracks,Samokutyayev Dock,Wundt Gateway,Vela Ring,Bethke Camp,Jemison Dock,Julian Gateway"
+ },
+ {
+ "name": "Flaming Star Sector LX-T b3-0",
+ "pos_x": -243.09375,
+ "pos_y": -77.5625,
+ "pos_z": -1687.71875,
+ "stations": "Flaming Star Logistics Centre"
+ },
+ {
+ "name": "Flech",
+ "pos_x": 20.125,
+ "pos_y": -10.34375,
+ "pos_z": -63.125,
+ "stations": "Escobar Ring,Stafford Terminal,Parry Terminal,Chargaff Terminal,Saberhagen Port,Burgess Gateway,Bergerac Base,Oxley Camp,Rescue Ship - Stafford Terminal"
+ },
+ {
+ "name": "Flechankob",
+ "pos_x": 20.6875,
+ "pos_y": -17.28125,
+ "pos_z": -162.84375,
+ "stations": "Acton Dock,Carter Orbital,Burroughs Arsenal"
+ },
+ {
+ "name": "Flechti",
+ "pos_x": 58.03125,
+ "pos_y": 46.34375,
+ "pos_z": 81.34375,
+ "stations": "Mastracchio Refinery,Nikitin Refinery,Rutherford Refinery"
+ },
+ {
+ "name": "Flectes",
+ "pos_x": 91.09375,
+ "pos_y": -163.21875,
+ "pos_z": 90.65625,
+ "stations": "Yerka Orbital,Geller Settlement,Mandel's Inheritance"
+ },
+ {
+ "name": "Flesk",
+ "pos_x": 34.625,
+ "pos_y": -87.34375,
+ "pos_z": 16.9375,
+ "stations": "Gregory Ryan Young,Glen's house of Lambada"
+ },
+ {
+ "name": "Flousop",
+ "pos_x": -1.625,
+ "pos_y": 16.8125,
+ "pos_z": 4,
+ "stations": "Irvin Refinery,Bosch Hangar,Salk Hub,Slade Base"
+ },
+ {
+ "name": "FN Virginis",
+ "pos_x": 7.5625,
+ "pos_y": 24.6875,
+ "pos_z": 6.15625,
+ "stations": "Jacobi Landing,Cantor Installation,Lorrah Barracks"
+ },
+ {
+ "name": "Fo Hi",
+ "pos_x": 41.1875,
+ "pos_y": -257.90625,
+ "pos_z": 60,
+ "stations": "Sukhoi Relay"
+ },
+ {
+ "name": "Fo Hsingin",
+ "pos_x": 10.28125,
+ "pos_y": -5.625,
+ "pos_z": 144.9375,
+ "stations": "Winne Colony,Grunsfeld Colony"
+ },
+ {
+ "name": "Folna",
+ "pos_x": 53.90625,
+ "pos_y": 77.9375,
+ "pos_z": 33.71875,
+ "stations": "Patsayev City,Lorentz Dock,Anderson Station,Schachner Prospect,Faris Orbital,Vela Station,Furukawa Dock,Smith Terminal,Cunningham Base,Gallun's Progress"
+ },
+ {
+ "name": "Fomalhaut",
+ "pos_x": -3.78125,
+ "pos_y": -22.875,
+ "pos_z": 9.6875,
+ "stations": "Wargnerport,Dobson Terminal,Cooke Station,Pavlov Asylum,Fort Lee,Gamow Depot,Dickensport,Baker Landing,Amis' Claim"
+ },
+ {
+ "name": "Fomonjekodi",
+ "pos_x": 52.1875,
+ "pos_y": -20.40625,
+ "pos_z": 168.0625,
+ "stations": "Pytheas Terminal,Weinbaum Camp"
+ },
+ {
+ "name": "Fomovithi",
+ "pos_x": -52.15625,
+ "pos_y": -34,
+ "pos_z": -84.625,
+ "stations": "Hale Hub,Padalka Terminal,Weyn Keep"
+ },
+ {
+ "name": "Fong Dadia",
+ "pos_x": 125.34375,
+ "pos_y": 72.21875,
+ "pos_z": 56.125,
+ "stations": "Blackwell Settlement,Brunel Enterprise,Bisson Observatory"
+ },
+ {
+ "name": "Fong Wang",
+ "pos_x": 58.03125,
+ "pos_y": 9.65625,
+ "pos_z": 36.03125,
+ "stations": "Wheelock Terminal,Schachner Dock,Volterra Hub,Barratt Vision,Polya Station"
+ },
+ {
+ "name": "Fonga",
+ "pos_x": 147.0625,
+ "pos_y": 3,
+ "pos_z": 18.125,
+ "stations": "Kafka Dock,MacCurdy Terminal,Lee Orbital,Pellegrino Installation"
+ },
+ {
+ "name": "Fongite",
+ "pos_x": 122.15625,
+ "pos_y": 114.0625,
+ "pos_z": -7.6875,
+ "stations": "Le Guin Mines,Cook Survey,Effinger Bastion"
+ },
+ {
+ "name": "Fongo",
+ "pos_x": 118.625,
+ "pos_y": -79.03125,
+ "pos_z": -23.4375,
+ "stations": "Flaugergues Reach"
+ },
+ {
+ "name": "Fongzi",
+ "pos_x": -51.59375,
+ "pos_y": -34.78125,
+ "pos_z": 151.40625,
+ "stations": "Zelazny Port,Gessi Prospect,Sekelj Works,Asire Enterprise"
+ },
+ {
+ "name": "Forculus",
+ "pos_x": -42.5625,
+ "pos_y": -24.03125,
+ "pos_z": 76.71875,
+ "stations": "Irrational Exuberance"
+ },
+ {
+ "name": "Forlog",
+ "pos_x": -52.03125,
+ "pos_y": 16.34375,
+ "pos_z": -82.21875,
+ "stations": "Bacon Terminal,Newton Platform"
+ },
+ {
+ "name": "Formanjia",
+ "pos_x": 47.09375,
+ "pos_y": -95.3125,
+ "pos_z": -53.75,
+ "stations": "Safdie Station,Ikeya Dock,Reichelt Platform"
+ },
+ {
+ "name": "Formod",
+ "pos_x": 40.71875,
+ "pos_y": 16.9375,
+ "pos_z": 140.28125,
+ "stations": "Boucher Survey,MacLeod Penal colony"
+ },
+ {
+ "name": "Formorians",
+ "pos_x": -30.75,
+ "pos_y": -32.78125,
+ "pos_z": 68.46875,
+ "stations": "Archambault Installation"
+ },
+ {
+ "name": "Formoring",
+ "pos_x": 77.21875,
+ "pos_y": -222.3125,
+ "pos_z": -61.28125,
+ "stations": "Mechain Hub,Qushji Dock,Jung City"
+ },
+ {
+ "name": "Forseti",
+ "pos_x": 59.625,
+ "pos_y": -143.875,
+ "pos_z": -47.625,
+ "stations": "Grigorovich Dock"
+ },
+ {
+ "name": "Forsha",
+ "pos_x": -44.40625,
+ "pos_y": 37.09375,
+ "pos_z": -124,
+ "stations": "Fung Survey"
+ },
+ {
+ "name": "Forsina",
+ "pos_x": 187.34375,
+ "pos_y": -146.15625,
+ "pos_z": 97.53125,
+ "stations": "Dunyach Relay,Zhu Terminal,McCrea Oasis,Very Bastion"
+ },
+ {
+ "name": "Fortuna",
+ "pos_x": -17.3125,
+ "pos_y": 15.875,
+ "pos_z": -54.46875,
+ "stations": "Snyder Market,McMonagle Gateway,Smith Terminal,Ericsson Landing,Abnett Base,Morris Arsenal,Leoniceno Terminal,Murray Market"
+ },
+ {
+ "name": "Fotla",
+ "pos_x": 64.78125,
+ "pos_y": -103.8125,
+ "pos_z": 43.09375,
+ "stations": "Ayers Orbital,Penzias Dock,Eskridge Beacon"
+ },
+ {
+ "name": "Fotlandjera",
+ "pos_x": 168,
+ "pos_y": -98.1875,
+ "pos_z": -47.5625,
+ "stations": "Arrhenius Colony,DiFate Depot"
+ },
+ {
+ "name": "Fousang",
+ "pos_x": 44.125,
+ "pos_y": -0.3125,
+ "pos_z": -12.78125,
+ "stations": "Nakaya Terminal,Akers Orbital,Midgley City,Gooch Barracks"
+ },
+ {
+ "name": "Frao",
+ "pos_x": 4.125,
+ "pos_y": -148.46875,
+ "pos_z": 123.75,
+ "stations": "Grunsfeld Town,Arzachel Survey,Korolev Station,Tarter Colony"
+ },
+ {
+ "name": "Frendan",
+ "pos_x": -14.25,
+ "pos_y": 29.65625,
+ "pos_z": 68.65625,
+ "stations": "Kandel Dock,Leiber Point,Adams Dock,Darboux Terminal"
+ },
+ {
+ "name": "Frendian",
+ "pos_x": -82.75,
+ "pos_y": 1.8125,
+ "pos_z": 66.0625,
+ "stations": "Santos Installation,Zebrowski Prospect"
+ },
+ {
+ "name": "Freng",
+ "pos_x": -74.9375,
+ "pos_y": -10.96875,
+ "pos_z": 54.125,
+ "stations": "Bear City,Pausch Relay,Werber Orbital,Keyes Hangar"
+ },
+ {
+ "name": "Frenis",
+ "pos_x": 43.28125,
+ "pos_y": -119.34375,
+ "pos_z": -95.4375,
+ "stations": "Shajn Dock,Capek Survey,Neumann Station,Parsons City,Hendel Settlement"
+ },
+ {
+ "name": "Frentani",
+ "pos_x": 83.1875,
+ "pos_y": -142.40625,
+ "pos_z": 107.4375,
+ "stations": "McMillan's Inheritance"
+ },
+ {
+ "name": "Frey",
+ "pos_x": -74.625,
+ "pos_y": -21.625,
+ "pos_z": 76.40625,
+ "stations": "Garratt Station,Crown Terminal"
+ },
+ {
+ "name": "Freyan",
+ "pos_x": 132.71875,
+ "pos_y": -40.5625,
+ "pos_z": 6.71875,
+ "stations": "Haro Orbital"
+ },
+ {
+ "name": "Fricassi",
+ "pos_x": 80.875,
+ "pos_y": -30.65625,
+ "pos_z": 18.09375,
+ "stations": "Armstrong Vision,Judson Prospect,Russ Point"
+ },
+ {
+ "name": "Frigah",
+ "pos_x": 119.21875,
+ "pos_y": -228.375,
+ "pos_z": 62.5,
+ "stations": "Bullialdus Platform,Eddington Bastion,Myasishchev Platform"
+ },
+ {
+ "name": "Frigaha",
+ "pos_x": -47.34375,
+ "pos_y": -0.15625,
+ "pos_z": 56.25,
+ "stations": "Aubakirov Orbital,Engle Orbital,Onizuka Landing,Green Keep"
+ },
+ {
+ "name": "Frigenses",
+ "pos_x": 116.03125,
+ "pos_y": 44,
+ "pos_z": 58.71875,
+ "stations": "Crown Mines"
+ },
+ {
+ "name": "Frigga",
+ "pos_x": -132.4375,
+ "pos_y": -24.4375,
+ "pos_z": 37.28125,
+ "stations": "Henson Ring,Bombelli Co-operative,Hovell Port,Danvers Holdings"
+ },
+ {
+ "name": "Friggi",
+ "pos_x": 84.96875,
+ "pos_y": -20.84375,
+ "pos_z": -68.96875,
+ "stations": "Bates Holdings,Donaldson Plant,Butcher Landing"
+ },
+ {
+ "name": "Friggirawi",
+ "pos_x": -112.21875,
+ "pos_y": 38.3125,
+ "pos_z": -19.875,
+ "stations": "Ivanishin Platform"
+ },
+ {
+ "name": "Frigh",
+ "pos_x": -59.125,
+ "pos_y": -2.78125,
+ "pos_z": 169.28125,
+ "stations": "von Krusenstern Reach"
+ },
+ {
+ "name": "Friguda",
+ "pos_x": 174.4375,
+ "pos_y": -156.9375,
+ "pos_z": 100.15625,
+ "stations": "Kaluta Base"
+ },
+ {
+ "name": "Frigur",
+ "pos_x": -4.09375,
+ "pos_y": -49.40625,
+ "pos_z": 80.75,
+ "stations": "Barba Hub,Illy Landing,Dickson Settlement,Gaultier de Varennes Base"
+ },
+ {
+ "name": "Frog",
+ "pos_x": -41.5,
+ "pos_y": -36.625,
+ "pos_z": 57.1875,
+ "stations": "Rochester,Kroehl Lab,Kempston Hardwick,Rasch Dock"
+ },
+ {
+ "name": "FS 34",
+ "pos_x": -24.8125,
+ "pos_y": -37.53125,
+ "pos_z": 50.5,
+ "stations": "Gorbatko Enterprise,Galton Landing"
+ },
+ {
+ "name": "FT Ceti",
+ "pos_x": -4.4375,
+ "pos_y": -64.21875,
+ "pos_z": -45.3125,
+ "stations": "Greenland City,Verrazzano Installation"
+ },
+ {
+ "name": "FT Piscium",
+ "pos_x": -25.5,
+ "pos_y": -23.6875,
+ "pos_z": -16.84375,
+ "stations": "Hawley City,Feynman City,Grunsfeld Port,Piper Installation,Ivins Horizons"
+ },
+ {
+ "name": "Fu Haiting",
+ "pos_x": -103,
+ "pos_y": 29.46875,
+ "pos_z": -43.4375,
+ "stations": "Walter Terminal,Hire Terminal,Bachman Depot,Ross Orbital"
+ },
+ {
+ "name": "Fu Haras",
+ "pos_x": 162.75,
+ "pos_y": -85.25,
+ "pos_z": 11.5625,
+ "stations": "Prandtl Port,Malvern Station,Bingzhen Hub,Fife Keep,King Vision,Almagro Vista"
+ },
+ {
+ "name": "Fu Hsi",
+ "pos_x": 126.03125,
+ "pos_y": -73.0625,
+ "pos_z": -125.1875,
+ "stations": "Evans Landing,Conti Prospect"
+ },
+ {
+ "name": "Fu Huacobad",
+ "pos_x": 159.75,
+ "pos_y": -112.5,
+ "pos_z": 44.3125,
+ "stations": "Humason Vision,Shinjo Vision,Hussenot Orbital,Hopkinson Vision"
+ },
+ {
+ "name": "Fu Huangaa",
+ "pos_x": 52.09375,
+ "pos_y": 44.65625,
+ "pos_z": 84.8125,
+ "stations": "Vizcaino Landing,Williams Landing,White Depot"
+ },
+ {
+ "name": "Fu Xianses",
+ "pos_x": 50.0625,
+ "pos_y": -46.6875,
+ "pos_z": 132,
+ "stations": "Benford Colony,Wegener Prospect,Hudson Refinery,Caselberg Station,Soto Terminal"
+ },
+ {
+ "name": "Fuambe",
+ "pos_x": 205.28125,
+ "pos_y": -182.9375,
+ "pos_z": 61.5625,
+ "stations": "Endate Vision,Reinhold Keep"
+ },
+ {
+ "name": "Fuamne",
+ "pos_x": -23.78125,
+ "pos_y": 117.96875,
+ "pos_z": 9.5,
+ "stations": "Corte-Real Settlement,Coelho Colony,Carpini Penal colony"
+ },
+ {
+ "name": "Fuamnoni",
+ "pos_x": -18.5625,
+ "pos_y": -138.28125,
+ "pos_z": 67.03125,
+ "stations": "Patry Holdings,Lloyd Wright Port"
+ },
+ {
+ "name": "Fuelum",
+ "pos_x": 52,
+ "pos_y": -52.65625,
+ "pos_z": 49.8125,
+ "stations": "Wollheim Vision,Plancius Penal colony,Carrasco Vision,Thesiger Enterprise,Padalka Colony"
+ },
+ {
+ "name": "Fugeirones",
+ "pos_x": -22.78125,
+ "pos_y": -44.28125,
+ "pos_z": 56.4375,
+ "stations": "Slade Settlement"
+ },
+ {
+ "name": "Fugeirs",
+ "pos_x": 133.40625,
+ "pos_y": 8.9375,
+ "pos_z": 5.78125,
+ "stations": "Crown Horizons,Norton Laboratory,Comer Point,Moffitt Gateway"
+ },
+ {
+ "name": "Fugen Nones",
+ "pos_x": 140.96875,
+ "pos_y": -1.21875,
+ "pos_z": 36.25,
+ "stations": "Nylund Plant,Brooks Settlement,Titius Station,De Lay Station,Zander Colony"
+ },
+ {
+ "name": "Fugen Shi",
+ "pos_x": 113.40625,
+ "pos_y": -177.1875,
+ "pos_z": 24.375,
+ "stations": "Abetti Station,Sukhoi Observatory,Tusi Point,Shavyrin Vision,Wolf Reach"
+ },
+ {
+ "name": "Fugeniez",
+ "pos_x": 4.21875,
+ "pos_y": 60.125,
+ "pos_z": -67.96875,
+ "stations": "Vasilyev Depot"
+ },
+ {
+ "name": "Fugenomo",
+ "pos_x": 0,
+ "pos_y": 103.25,
+ "pos_z": -50.65625,
+ "stations": "Moon's Claim,Robinson Orbital"
+ },
+ {
+ "name": "Fugeth",
+ "pos_x": 83.84375,
+ "pos_y": 9.3125,
+ "pos_z": 87.28125,
+ "stations": "Hooke Colony,Schirra Orbital,Wang Survey"
+ },
+ {
+ "name": "Fujin",
+ "pos_x": -6.03125,
+ "pos_y": -30.375,
+ "pos_z": -59.03125,
+ "stations": "Futen Spaceport"
+ },
+ {
+ "name": "Fular",
+ "pos_x": 4.3125,
+ "pos_y": -18.03125,
+ "pos_z": -81.25,
+ "stations": "Marshburn Installation,Kotov Orbital,Clifton Vision,MacLean Horizons"
+ },
+ {
+ "name": "Fulastobo",
+ "pos_x": 3.53125,
+ "pos_y": -20.59375,
+ "pos_z": 111,
+ "stations": "Crampton Orbital,Kregel Port,Haber Port,Lebedev Station,Shuttleworth Orbital,Currie Station,Schwann Port,Chilton Port,Blaha Orbital,Margulies Enterprise,Stross Landing,Dann's Progress,Schweickart Installation,Andrews Survey"
+ },
+ {
+ "name": "Fullabog",
+ "pos_x": 124.90625,
+ "pos_y": 28.96875,
+ "pos_z": 103.96875,
+ "stations": "Puleston Colony"
+ },
+ {
+ "name": "Fullerene C60",
+ "pos_x": -50.5,
+ "pos_y": 19.28125,
+ "pos_z": -203.875,
+ "stations": "Rebuy Prospect"
+ },
+ {
+ "name": "Fullich",
+ "pos_x": -124,
+ "pos_y": 48.4375,
+ "pos_z": 60.8125,
+ "stations": "Viete Platform,Ali Point"
+ },
+ {
+ "name": "Funj",
+ "pos_x": -37.9375,
+ "pos_y": -75.90625,
+ "pos_z": -16.25,
+ "stations": "Cauchy Ring"
+ },
+ {
+ "name": "Funji",
+ "pos_x": -119.0625,
+ "pos_y": 15.125,
+ "pos_z": -113.25,
+ "stations": "Stein Platform"
+ },
+ {
+ "name": "Funjila",
+ "pos_x": 89.53125,
+ "pos_y": -93.40625,
+ "pos_z": -87.46875,
+ "stations": "Delsanti Orbital,Neugebauer City,Siegel Station,Weber Keep"
+ },
+ {
+ "name": "Furbaide",
+ "pos_x": -28.5625,
+ "pos_y": -10.65625,
+ "pos_z": 28.8125,
+ "stations": "Walker Point,Effinger Port,Cook Terminal,Griggs Installation"
+ },
+ {
+ "name": "Furuhjelm I-645",
+ "pos_x": -11.71875,
+ "pos_y": 92.125,
+ "pos_z": -45.6875,
+ "stations": "Gidzenko Ring"
+ },
+ {
+ "name": "Furuhjelm II-432",
+ "pos_x": -5.03125,
+ "pos_y": 26.40625,
+ "pos_z": -81.28125,
+ "stations": "Slade Colony,Hale's Inheritance"
+ },
+ {
+ "name": "Furuhjelm III-674",
+ "pos_x": -39,
+ "pos_y": -8.125,
+ "pos_z": -87.75,
+ "stations": "Tshang City,Fadlan Port,Scalzi Base,Knight Plant,Barnes Hub"
+ },
+ {
+ "name": "Fusang",
+ "pos_x": -12.59375,
+ "pos_y": -19.03125,
+ "pos_z": 39.71875,
+ "stations": "Cleve Vision,Cook Installation,Zoline Vision"
+ },
+ {
+ "name": "Futeno",
+ "pos_x": -32.71875,
+ "pos_y": 5.1875,
+ "pos_z": 166.3125,
+ "stations": "Haipeng Ring,Hermaszewski Installation,McCandless Prospect,Drzewiecki Survey,Coelho Hub"
+ },
+ {
+ "name": "Futes",
+ "pos_x": 61.5,
+ "pos_y": -77.8125,
+ "pos_z": -20.4375,
+ "stations": "Porco Platform,DiFate Prospect,Tanaka Hub,Burkin Installation,Effinger Prospect"
+ },
+ {
+ "name": "Futhark",
+ "pos_x": 75.34375,
+ "pos_y": -119.28125,
+ "pos_z": 46.4375,
+ "stations": "Barker's Horizon,Gorey Port,Ray Depot,Eddington's Inheritance,Jeury Port,Ravescene Memorial,Wigura City"
+ },
+ {
+ "name": "Futhorc",
+ "pos_x": 80.5625,
+ "pos_y": -122.0625,
+ "pos_z": 45.09375,
+ "stations": "Benlivia,Acquired Taste Orbital,Neujmin Hub,Dassault's Progress,Giancola Horizons"
+ },
+ {
+ "name": "FW Cephei",
+ "pos_x": -1415.78125,
+ "pos_y": 366.65625,
+ "pos_z": -355.3125,
+ "stations": "Iris Vacations"
+ },
+ {
+ "name": "Fyrly",
+ "pos_x": 15.0625,
+ "pos_y": -79.78125,
+ "pos_z": 34.09375,
+ "stations": "Boming Point,Buckey Settlement,Herschel City"
+ },
+ {
+ "name": "G 107-65",
+ "pos_x": -6.59375,
+ "pos_y": 22.625,
+ "pos_z": -48.0625,
+ "stations": "Brand Mines"
+ },
+ {
+ "name": "G 108-26",
+ "pos_x": 30.0625,
+ "pos_y": 0.25,
+ "pos_z": -51.625,
+ "stations": "Ejeta Colony,Moore Bastion,Stuart Vision"
+ },
+ {
+ "name": "G 109-55",
+ "pos_x": 7.3125,
+ "pos_y": 12.21875,
+ "pos_z": -37.65625,
+ "stations": "Schlegel City,Grabe Refinery,Rushworth Settlement,Crown Holdings,Adams Landing"
+ },
+ {
+ "name": "G 113-20",
+ "pos_x": 18.78125,
+ "pos_y": 10.09375,
+ "pos_z": -20.75,
+ "stations": "Cernan Lab,Carson Settlement,Ansari Hangar,Faris Survey"
+ },
+ {
+ "name": "G 116-72",
+ "pos_x": -3.5,
+ "pos_y": 56.875,
+ "pos_z": -44.78125,
+ "stations": "Dias Works,Adams Colony,Janszoon Station"
+ },
+ {
+ "name": "G 121-8",
+ "pos_x": 9.1875,
+ "pos_y": 58.75,
+ "pos_z": -10.59375,
+ "stations": "Kapp Vision,Spielberg Dock,Nylund Horizons"
+ },
+ {
+ "name": "G 122-60",
+ "pos_x": -11,
+ "pos_y": 73.46875,
+ "pos_z": -22.5625,
+ "stations": "Low Hub,Watts Enterprise,Vancouver Gateway,Baker Vision"
+ },
+ {
+ "name": "G 123-16",
+ "pos_x": -9.25,
+ "pos_y": 79.03125,
+ "pos_z": -17.75,
+ "stations": "Anthony de la Roche Gateway,Shiner Station,Shosuke Silo,Koontz Installation,Mieville Holdings"
+ },
+ {
+ "name": "G 123-49",
+ "pos_x": -18.90625,
+ "pos_y": 66.875,
+ "pos_z": -14.78125,
+ "stations": "Herreshoff Freeport,Besonders Landing,Daley Prospect"
+ },
+ {
+ "name": "G 123-7",
+ "pos_x": -9.59375,
+ "pos_y": 77.1875,
+ "pos_z": -18.6875,
+ "stations": "Rubruck Refinery,Burgess Refinery"
+ },
+ {
+ "name": "G 125-30",
+ "pos_x": -34.46875,
+ "pos_y": 2.3125,
+ "pos_z": 13.96875,
+ "stations": "Popov Installation,Francisco de Almeida Bastion"
+ },
+ {
+ "name": "G 126-31",
+ "pos_x": -48.5625,
+ "pos_y": -25.6875,
+ "pos_z": 16.0625,
+ "stations": "Dobrovolski Ring,Soukup Prospect,Nikitin Relay"
+ },
+ {
+ "name": "G 130-9",
+ "pos_x": -53.78125,
+ "pos_y": -26.09375,
+ "pos_z": -18.5625,
+ "stations": "Hughes Station"
+ },
+ {
+ "name": "G 138-40",
+ "pos_x": -19.25,
+ "pos_y": 31.3125,
+ "pos_z": 40.03125,
+ "stations": "Leonov Dock,Thorne Hub,Herrington Station,Kuipers Arsenal,Simak Point"
+ },
+ {
+ "name": "G 139-21",
+ "pos_x": -17.03125,
+ "pos_y": 16.875,
+ "pos_z": 34.625,
+ "stations": "Stith Port,Bixby Port,Fleming Vision,Dirichlet Works,Bethke Ring"
+ },
+ {
+ "name": "G 139-3",
+ "pos_x": -19.90625,
+ "pos_y": 21.96875,
+ "pos_z": 30.25,
+ "stations": "O'Connor Dock,Swanson Plant,Marshburn Hub"
+ },
+ {
+ "name": "G 139-50",
+ "pos_x": -33.84375,
+ "pos_y": 22.96875,
+ "pos_z": 54.25,
+ "stations": "Filipchenko City,Schweinfurth Platform,Kondratyev Dock,Schweickart Holdings"
+ },
+ {
+ "name": "G 14-6",
+ "pos_x": 13.5,
+ "pos_y": 30.375,
+ "pos_z": 9.0625,
+ "stations": "Daimler Observatory,Humboldt Dock,Knipling Refinery,Burroughs Base"
+ },
+ {
+ "name": "G 140-9",
+ "pos_x": -31.625,
+ "pos_y": 19.34375,
+ "pos_z": 53.5,
+ "stations": "Anning Platform,Artsebarsky Port,Chomsky Landing,Hasse Holdings,Hopkins Penal colony"
+ },
+ {
+ "name": "G 141-21",
+ "pos_x": -24.875,
+ "pos_y": 6,
+ "pos_z": 26.21875,
+ "stations": "McDaniel Terminal,Scithers Hub,Amnuel Orbital,Kimberlin Enterprise,Piercy Terminal,Sekelj Station,Aldiss Vision,Ryazanski Beacon,Alexeyev Hub,Willis Point,White Enterprise,Beltrami Mines,Crick Vision,Cousteau Beacon"
+ },
+ {
+ "name": "G 141-52",
+ "pos_x": -32.9375,
+ "pos_y": 19.0625,
+ "pos_z": 30.125,
+ "stations": "Chilton Co-operative,Clairaut Port,Pournelle Station"
+ },
+ {
+ "name": "G 146-5",
+ "pos_x": -7.375,
+ "pos_y": 53.9375,
+ "pos_z": -43.03125,
+ "stations": "vo Survey,Ampere Survey,Grover Mines"
+ },
+ {
+ "name": "G 165-13",
+ "pos_x": -13.15625,
+ "pos_y": 71.5,
+ "pos_z": 5.65625,
+ "stations": "Naddoddur Platform,Hausdorff Station"
+ },
+ {
+ "name": "G 166-21",
+ "pos_x": -16.40625,
+ "pos_y": 71.3125,
+ "pos_z": 19.90625,
+ "stations": "Bokeili Dock,Lagrange Dock,Haarsma Vision,Kotov Depot"
+ },
+ {
+ "name": "G 167-35",
+ "pos_x": -33.15625,
+ "pos_y": 71.9375,
+ "pos_z": 32.9375,
+ "stations": "Bujold Vision"
+ },
+ {
+ "name": "G 172-13",
+ "pos_x": -46.46875,
+ "pos_y": -16.71875,
+ "pos_z": -26.96875,
+ "stations": "Kadenyuk Terminal,Schmitz Terminal,Ryazanski Refinery"
+ },
+ {
+ "name": "G 173-53",
+ "pos_x": -25.15625,
+ "pos_y": -8.03125,
+ "pos_z": -28.65625,
+ "stations": "Polansky Hub,McArthur Landing,Shukor Point,Cooper Settlement,Wedge's Folly"
+ },
+ {
+ "name": "G 175-42",
+ "pos_x": -14.65625,
+ "pos_y": 2.5,
+ "pos_z": -29.46875,
+ "stations": "Werber Platform,Coke Vista"
+ },
+ {
+ "name": "G 176-29",
+ "pos_x": -13.375,
+ "pos_y": 74.5625,
+ "pos_z": -33.6875,
+ "stations": "Serling Terminal,Jones Beacon"
+ },
+ {
+ "name": "G 180-18",
+ "pos_x": -28.96875,
+ "pos_y": 40.6875,
+ "pos_z": 19.03125,
+ "stations": "Hale Port,Bamford Terminal,Lovell City,Krylov Installation,Vizcaino Beacon"
+ },
+ {
+ "name": "G 180-47",
+ "pos_x": -51.84375,
+ "pos_y": 65.5625,
+ "pos_z": 40.8125,
+ "stations": "Rothman Bastion,McCulley Hub"
+ },
+ {
+ "name": "G 181-6",
+ "pos_x": -20.3125,
+ "pos_y": 20.3125,
+ "pos_z": 14.0625,
+ "stations": "Scheutz Hub,Polansky Enterprise,Pavlov Hub,Robinson Keep,Eisele Beacon"
+ },
+ {
+ "name": "G 188-9",
+ "pos_x": -40.53125,
+ "pos_y": -13.65625,
+ "pos_z": 7.59375,
+ "stations": "Gubarev City,Charnas Bastion,Tanner Dock,Velazquez Reformatory"
+ },
+ {
+ "name": "G 190-28",
+ "pos_x": -44,
+ "pos_y": -15.65625,
+ "pos_z": -13.3125,
+ "stations": "Guidoni Dock,Kooi Gateway,Schiltberger Installation,Townshend Enterprise,Aldrin Base"
+ },
+ {
+ "name": "G 203-47",
+ "pos_x": -18.34375,
+ "pos_y": 14.25,
+ "pos_z": 7.09375,
+ "stations": "Akers Enterprise,Cabana Market,Thorne Market,Ride Gateway,Gantt Arsenal,Siemens Camp"
+ },
+ {
+ "name": "G 203-51",
+ "pos_x": -15.875,
+ "pos_y": 12.03125,
+ "pos_z": 5.34375,
+ "stations": "The Shield of Resolve"
+ },
+ {
+ "name": "G 205-47",
+ "pos_x": -63.96875,
+ "pos_y": 21.96875,
+ "pos_z": 15.59375,
+ "stations": "Babcock Base,Bain Horizons"
+ },
+ {
+ "name": "G 214-14",
+ "pos_x": -72.28125,
+ "pos_y": -16,
+ "pos_z": -4.5625,
+ "stations": "Redi Point,Gauss Terminal"
+ },
+ {
+ "name": "G 218-5",
+ "pos_x": -64.96875,
+ "pos_y": -13.75,
+ "pos_z": -39.5625,
+ "stations": "Cooper Platform,Tani Orbital"
+ },
+ {
+ "name": "G 224-46",
+ "pos_x": -55.25,
+ "pos_y": 60.34375,
+ "pos_z": -12.71875,
+ "stations": "Zebrowski Ring,Pierce Port,Broderick's Progress,Rozhdestvensky Base,Lorrah Dock"
+ },
+ {
+ "name": "G 230-27",
+ "pos_x": -58.125,
+ "pos_y": 11.4375,
+ "pos_z": 2.6875,
+ "stations": "Laplace Works"
+ },
+ {
+ "name": "G 232-62",
+ "pos_x": -67.96875,
+ "pos_y": -2.03125,
+ "pos_z": -14.03125,
+ "stations": "Halley Installation,Hauck Settlement,Borman Station"
+ },
+ {
+ "name": "G 239-25",
+ "pos_x": -22.6875,
+ "pos_y": 25.8125,
+ "pos_z": -6.6875,
+ "stations": "Bresnik Mine,Magnus Forum,Moore Forum"
+ },
+ {
+ "name": "G 240-11",
+ "pos_x": -74.21875,
+ "pos_y": 67.34375,
+ "pos_z": -2.375,
+ "stations": "Smith Ring,Bailey Hub,Walker Hub,Meredith Gateway,Thompson Gateway,Asher Station,Lehtonen Enterprise"
+ },
+ {
+ "name": "G 250-34",
+ "pos_x": -27.28125,
+ "pos_y": 25.90625,
+ "pos_z": -44.5,
+ "stations": "Porges Horizons,Macan Hub,Martin Ring,Bond Silo,Kozin Beacon"
+ },
+ {
+ "name": "G 266-46",
+ "pos_x": -18.5625,
+ "pos_y": -161.65625,
+ "pos_z": 19.84375,
+ "stations": "Grigorovich Terminal,Fancher Base,Binney Oasis"
+ },
+ {
+ "name": "G 267-2",
+ "pos_x": -8.40625,
+ "pos_y": -161.125,
+ "pos_z": 31.96875,
+ "stations": "Bradley Orbital,Chelomey City,Thierree Dock"
+ },
+ {
+ "name": "G 267-24",
+ "pos_x": 7.90625,
+ "pos_y": -163.09375,
+ "pos_z": 35.875,
+ "stations": "Vogel Hub,Beer Dock,Schiltberger Prospect,Shunkai Port,Szameit Works"
+ },
+ {
+ "name": "G 268-47",
+ "pos_x": -3.78125,
+ "pos_y": -60.28125,
+ "pos_z": -1.21875,
+ "stations": "Archambault Hub,Landsteiner Orbital,Dyson Ring,Navigator Enterprise,Speke Enterprise"
+ },
+ {
+ "name": "G 275-50",
+ "pos_x": -7.40625,
+ "pos_y": -150.40625,
+ "pos_z": 49.9375,
+ "stations": "Zander Beacon,Korolyov Point,Sturgeon Keep"
+ },
+ {
+ "name": "G 30-26",
+ "pos_x": -36.0625,
+ "pos_y": -48.21875,
+ "pos_z": -4.96875,
+ "stations": "Lopez de Haro's Folly,Frick Port,Boltzmann Hangar"
+ },
+ {
+ "name": "G 35-15",
+ "pos_x": -28.3125,
+ "pos_y": -36.9375,
+ "pos_z": -35.0625,
+ "stations": "Leslie Gateway,Tereshkova Hub,Aleksandrov Terminal,Yegorov Ring,Cugnot Terminal,Kirchoff Hub,Barr Legacy,Morgan Legacy,Cardano Gateway,Hobaugh Station,Mendeleev Hub,Oluwafemi Terminal,Weitz's Folly,Payette Landing"
+ },
+ {
+ "name": "G 41-14",
+ "pos_x": 7.9375,
+ "pos_y": 7.71875,
+ "pos_z": -9.4375,
+ "stations": "Ramon Hub"
+ },
+ {
+ "name": "G 5-32",
+ "pos_x": -7.09375,
+ "pos_y": -35.40625,
+ "pos_z": -47.34375,
+ "stations": "Hunt Silo,Andreas Hangar,Halsell Orbital,Kovalyonok Landing,Gardner Survey"
+ },
+ {
+ "name": "G 59-7",
+ "pos_x": 8.90625,
+ "pos_y": 78.625,
+ "pos_z": -8.09375,
+ "stations": "Drake Plant,Lagrange City,Hopkins Colony,Cook's Progress"
+ },
+ {
+ "name": "G 65-9",
+ "pos_x": 9.53125,
+ "pos_y": 42.34375,
+ "pos_z": 20.34375,
+ "stations": "Mourelle Gateway,MacCurdy Hub,Strzelecki's Progress,Hume Orbital"
+ },
+ {
+ "name": "G 69-11",
+ "pos_x": -45.34375,
+ "pos_y": -32.09375,
+ "pos_z": -26.5,
+ "stations": "Cadamosto Prospect"
+ },
+ {
+ "name": "G 83-13",
+ "pos_x": 1.21875,
+ "pos_y": -16.09375,
+ "pos_z": -38.03125,
+ "stations": "Bowen Dock,Ivanchenkov Terminal,Agnesi City,Pawelczyk Port,Schrodinger Dock,Burbank Dock,Rushworth Base,Asaro Relay,Bohm City,Nagel City,Carr Port"
+ },
+ {
+ "name": "G 85-12",
+ "pos_x": -5.03125,
+ "pos_y": -8.53125,
+ "pos_z": -41.21875,
+ "stations": "Bhabha City,Garn Colony,Great Survey"
+ },
+ {
+ "name": "G 87-16",
+ "pos_x": 5.90625,
+ "pos_y": 15.6875,
+ "pos_z": -57.5,
+ "stations": "Brackett Orbital,Ostrander Station,Ashton Station,Seega Installation,Belyanin Orbital,Clayton Port,MacLean Port,Elvstrom City,Silverberg Dock,Makarov Prospect,Henderson Dock,Liouville Gateway"
+ },
+ {
+ "name": "G 89-32",
+ "pos_x": 10.28125,
+ "pos_y": 4.59375,
+ "pos_z": -16.6875,
+ "stations": "Conrad Holdings,Hopper Vision"
+ },
+ {
+ "name": "G 97-54",
+ "pos_x": 7.96875,
+ "pos_y": -7.03125,
+ "pos_z": -39,
+ "stations": "Aksyonov Terminal,Kekule Hub,Ford Installation"
+ },
+ {
+ "name": "G 98-44",
+ "pos_x": -2.34375,
+ "pos_y": 8.71875,
+ "pos_z": -79.0625,
+ "stations": "Oswald Ring,Glashow Landing,Gelfand Relay,Wankel Terminal"
+ },
+ {
+ "name": "G 99-49",
+ "pos_x": 7.15625,
+ "pos_y": -3.09375,
+ "pos_z": -15.6875,
+ "stations": "Linnaeus Station,Willis Landing,Carlisle Vision,Noguchi Arsenal"
+ },
+ {
+ "name": "g Eridani",
+ "pos_x": 111.5,
+ "pos_y": -163.1875,
+ "pos_z": -70.6875,
+ "stations": "Kanai Vision,Frazetta Prospect"
+ },
+ {
+ "name": "G Hydrae",
+ "pos_x": 140.71875,
+ "pos_y": 54.5,
+ "pos_z": -41.71875,
+ "stations": "Boming Port,Carpenter Terminal,Laplace Port,Anthony de la Roche Relay"
+ },
+ {
+ "name": "G Scorpii",
+ "pos_x": 13.4375,
+ "pos_y": -12.15625,
+ "pos_z": 124.5,
+ "stations": "Flint City,Roberts Ring,Wolfe Hub,Shirley Bastion,Hoffman Prospect"
+ },
+ {
+ "name": "G146-60",
+ "pos_x": -12.75,
+ "pos_y": 73.65625,
+ "pos_z": -44.21875,
+ "stations": "Lee Hub,Pellegrino Landing,Greenleaf Co-operative"
+ },
+ {
+ "name": "Ga Gaun",
+ "pos_x": -8.09375,
+ "pos_y": 77.875,
+ "pos_z": -55.96875,
+ "stations": "Taine Holdings"
+ },
+ {
+ "name": "Ga Gautas",
+ "pos_x": 49.375,
+ "pos_y": -128.1875,
+ "pos_z": -55.53125,
+ "stations": "Tupolev Port,Sheremetevsky Colony"
+ },
+ {
+ "name": "Ga Gigah",
+ "pos_x": -50.8125,
+ "pos_y": -67.25,
+ "pos_z": -120.6875,
+ "stations": "Fullerton Point,Buchli Settlement,Smith Orbital"
+ },
+ {
+ "name": "Ga Gu",
+ "pos_x": 32.03125,
+ "pos_y": -102.625,
+ "pos_z": -76.34375,
+ "stations": "Henson Silo,Fernandes Dock,Holub Camp"
+ },
+ {
+ "name": "Ga Ohkambo",
+ "pos_x": 79.46875,
+ "pos_y": -160.65625,
+ "pos_z": 136.59375,
+ "stations": "Lalande Settlement,Pond Terminal,Bernoulli Base"
+ },
+ {
+ "name": "Ga Okya",
+ "pos_x": 93.65625,
+ "pos_y": -98.1875,
+ "pos_z": -90.5625,
+ "stations": "Eisinga Settlement,Schroter Vision"
+ },
+ {
+ "name": "Ga'anjob'al",
+ "pos_x": 78.5,
+ "pos_y": 24.59375,
+ "pos_z": -10.34375,
+ "stations": "Wellman Station,Acaba Dock,Anderson Lab"
+ },
+ {
+ "name": "Ga'at",
+ "pos_x": 132.9375,
+ "pos_y": -70.03125,
+ "pos_z": 11.0625,
+ "stations": "Jewitt Port,Hardy Station,Bogdanov Penal colony,Arp Vision,Jean Gateway,O'Neill Orbital,Petaja Prospect,Chwedyk's Folly"
+ },
+ {
+ "name": "Gaantenoi",
+ "pos_x": 88.1875,
+ "pos_y": 30.3125,
+ "pos_z": 103.03125,
+ "stations": "Popovich Legacy"
+ },
+ {
+ "name": "Gaarnae",
+ "pos_x": 12.875,
+ "pos_y": -177.09375,
+ "pos_z": 92.65625,
+ "stations": "Roth Beacon,Shoemaker Horizons"
+ },
+ {
+ "name": "Gaarnakuruk",
+ "pos_x": 89.40625,
+ "pos_y": -256,
+ "pos_z": 50.40625,
+ "stations": "Thierree Port"
+ },
+ {
+ "name": "Gaarnuten",
+ "pos_x": 12.125,
+ "pos_y": -101.84375,
+ "pos_z": 139.53125,
+ "stations": "Sternbach Vision,van den Bergh Ring,Salpeter Dock,Greenleaf Horizons,Saberhagen Base"
+ },
+ {
+ "name": "Gabia",
+ "pos_x": -21.3125,
+ "pos_y": -79.46875,
+ "pos_z": -144.125,
+ "stations": "Lee Horizons,Kippax City,Bacigalupi Base"
+ },
+ {
+ "name": "Gabien Ku",
+ "pos_x": -58.5,
+ "pos_y": -70.0625,
+ "pos_z": -13.78125,
+ "stations": "Landsteiner Installation,Braun Beacon"
+ },
+ {
+ "name": "Gabietye",
+ "pos_x": -29.84375,
+ "pos_y": -62.875,
+ "pos_z": 61.9375,
+ "stations": "Holberg Terminal"
+ },
+ {
+ "name": "Gabihilota",
+ "pos_x": -141.1875,
+ "pos_y": -16.875,
+ "pos_z": 12.40625,
+ "stations": "McMonagle Station"
+ },
+ {
+ "name": "Gabiko",
+ "pos_x": -22.0625,
+ "pos_y": -152.4375,
+ "pos_z": 26.46875,
+ "stations": "Yamamoto Enterprise,Carleson's Folly,Willis Hub"
+ },
+ {
+ "name": "Gabikula",
+ "pos_x": -34.40625,
+ "pos_y": -82.6875,
+ "pos_z": -104.5,
+ "stations": "Galindo Reformatory"
+ },
+ {
+ "name": "Gabin",
+ "pos_x": 62.09375,
+ "pos_y": 34.8125,
+ "pos_z": -89.59375,
+ "stations": "Shipton Terminal,Mille Refinery,Abernathy Port,Crook Port,Alexeyev Terminal"
+ },
+ {
+ "name": "Gabjaujavas",
+ "pos_x": -97.875,
+ "pos_y": 38.59375,
+ "pos_z": 37.34375,
+ "stations": "Vetulani Installation"
+ },
+ {
+ "name": "Gabjaujini",
+ "pos_x": -94.9375,
+ "pos_y": 57.90625,
+ "pos_z": 117.65625,
+ "stations": "Rothman Landing,Laing Terminal,Carleson Enterprise"
+ },
+ {
+ "name": "Gabjaujis",
+ "pos_x": 144.625,
+ "pos_y": -120.375,
+ "pos_z": 63.84375,
+ "stations": "Tsiolkovsky Terminal,Sopwith Port,Eddington Station,Dugan City"
+ },
+ {
+ "name": "Gabraceni",
+ "pos_x": 52.53125,
+ "pos_y": -11.375,
+ "pos_z": 61.65625,
+ "stations": "Stanley Station,Patterson Terminal,Hughes Market,Ahern Ring,Bentham Barracks"
+ },
+ {
+ "name": "Gabrani",
+ "pos_x": -112.1875,
+ "pos_y": 9.4375,
+ "pos_z": 52.78125,
+ "stations": "Janifer Base,Mohri Settlement,Fung Landing"
+ },
+ {
+ "name": "Gabri",
+ "pos_x": -34.71875,
+ "pos_y": 199.875,
+ "pos_z": -101.03125,
+ "stations": "Tull Station,Ron Hubbard Hub,Svavarsson Horizons,Mastracchio Landing,Carson Station"
+ },
+ {
+ "name": "Gabriges",
+ "pos_x": -21.25,
+ "pos_y": -18.90625,
+ "pos_z": 95.84375,
+ "stations": "Melnick Landing,Forest Landing,Smith Settlement,Ramsbottom Point"
+ },
+ {
+ "name": "Gabriguri",
+ "pos_x": 173.21875,
+ "pos_y": 0.84375,
+ "pos_z": 48.25,
+ "stations": "McQuay Vision"
+ },
+ {
+ "name": "Gacrux",
+ "pos_x": 75.90625,
+ "pos_y": 8.3125,
+ "pos_z": 44.8125,
+ "stations": "Ramanujan Terminal,Vaucanson Orbital,Chiao Arsenal,Schrodinger Dock,Fairbairn Station,Slayton Camp,Hopkinson's Inheritance,Melroy Beacon"
+ },
+ {
+ "name": "Gadagese",
+ "pos_x": -43.84375,
+ "pos_y": 145.09375,
+ "pos_z": 19.1875,
+ "stations": "Menezes Prospect"
+ },
+ {
+ "name": "Gadagombiri",
+ "pos_x": 97.3125,
+ "pos_y": 20.625,
+ "pos_z": 77.71875,
+ "stations": "Gooch Survey,Frimout Stop,Hutchinson Settlement"
+ },
+ {
+ "name": "Gadagua",
+ "pos_x": -118.5625,
+ "pos_y": 68.6875,
+ "pos_z": -13.40625,
+ "stations": "Szebehely Beacon,Rushd Settlement"
+ },
+ {
+ "name": "Gadaninovii",
+ "pos_x": 31.59375,
+ "pos_y": -47.03125,
+ "pos_z": 128.78125,
+ "stations": "Clark Terminal,Sturt Reach,Hadfield Station"
+ },
+ {
+ "name": "Gadjali",
+ "pos_x": 106.5625,
+ "pos_y": 15.34375,
+ "pos_z": 25.71875,
+ "stations": "Hughes Port,Cook Survey"
+ },
+ {
+ "name": "Gadjil",
+ "pos_x": -116.71875,
+ "pos_y": 46.78125,
+ "pos_z": 91.8125,
+ "stations": "Bradbury Landing,Shelley Settlement,Silves Holdings,Stafford Landing"
+ },
+ {
+ "name": "Gaezani",
+ "pos_x": 142.15625,
+ "pos_y": -216.34375,
+ "pos_z": 35.65625,
+ "stations": "Rees Terminal,Fieseler Vista"
+ },
+ {
+ "name": "Gaezatorix",
+ "pos_x": -0.78125,
+ "pos_y": -74.375,
+ "pos_z": -149.15625,
+ "stations": "Heyerdahl Depot,Brandenstein Beacon,Darwin Dock"
+ },
+ {
+ "name": "Gakia",
+ "pos_x": 95.90625,
+ "pos_y": 103.0625,
+ "pos_z": -89.78125,
+ "stations": "Khan Dock,Caidin Bastion"
+ },
+ {
+ "name": "Gakimo",
+ "pos_x": -75.3125,
+ "pos_y": -61.0625,
+ "pos_z": 85,
+ "stations": "Williams Settlement,Baffin Installation,Teng-hui Installation"
+ },
+ {
+ "name": "Gakiutl",
+ "pos_x": -19.46875,
+ "pos_y": -36.3125,
+ "pos_z": 67.15625,
+ "stations": "Bykovsky Orbital"
+ },
+ {
+ "name": "Galam",
+ "pos_x": 2.71875,
+ "pos_y": -123.96875,
+ "pos_z": -65.46875,
+ "stations": "Saarinen Dock,Rosseland Vision,Russell Holdings"
+ },
+ {
+ "name": "Galandandes",
+ "pos_x": 46.3125,
+ "pos_y": -119.1875,
+ "pos_z": 64.0625,
+ "stations": "Schroter Colony,Anderson Landing,Herodotus Settlement"
+ },
+ {
+ "name": "Galapana",
+ "pos_x": -93.75,
+ "pos_y": 80.03125,
+ "pos_z": -16.75,
+ "stations": "Diesel Station,Overmyer Hub,Vinci Enterprise,Dayuan Hub"
+ },
+ {
+ "name": "Gali",
+ "pos_x": -57.625,
+ "pos_y": 43.40625,
+ "pos_z": 97.5625,
+ "stations": "Ostwald Station,Kaleri Terminal,Chadwick Dock,Kregel Hub,Nakaya Hub,Patsayev Dock,Serebrov Hub,Clement Forum,Hoyle Forum"
+ },
+ {
+ "name": "Galibi",
+ "pos_x": 37.09375,
+ "pos_y": -2.4375,
+ "pos_z": -89.03125,
+ "stations": "Ciferri Dock"
+ },
+ {
+ "name": "Galis",
+ "pos_x": 163.90625,
+ "pos_y": -23.375,
+ "pos_z": -99.0625,
+ "stations": "Cantor Hub,Herreshoff Keep,Semeonis Vision"
+ },
+ {
+ "name": "Galit",
+ "pos_x": -49.125,
+ "pos_y": 96.65625,
+ "pos_z": -110.6875,
+ "stations": "Jordan Dock,Speke Hub,Gotlieb Survey"
+ },
+ {
+ "name": "Galitini",
+ "pos_x": 21.15625,
+ "pos_y": 113.90625,
+ "pos_z": -34.84375,
+ "stations": "Wafer Outpost,Stillman Estate,Clarke Terminal"
+ },
+ {
+ "name": "Gallaecian",
+ "pos_x": 3.09375,
+ "pos_y": 65.65625,
+ "pos_z": 103.5,
+ "stations": "Kolmogorov Platform,Oxley's Folly"
+ },
+ {
+ "name": "Gallaeni",
+ "pos_x": 170.25,
+ "pos_y": -160.3125,
+ "pos_z": 84.375,
+ "stations": "Grushin Legacy,Dixon Dock"
+ },
+ {
+ "name": "Gallauni",
+ "pos_x": -25.46875,
+ "pos_y": -142.1875,
+ "pos_z": -75.15625,
+ "stations": "Charlier Hub,Alexandria Station,Powell Installation"
+ },
+ {
+ "name": "Gallavs",
+ "pos_x": -33.09375,
+ "pos_y": -22.34375,
+ "pos_z": -130.25,
+ "stations": "Aksyonov Arsenal,Hume Lab,Scott Hub,Haller Platform"
+ },
+ {
+ "name": "Gallemi",
+ "pos_x": 68.1875,
+ "pos_y": -262.125,
+ "pos_z": -5.5625,
+ "stations": "Rabinowitz Vision,Norman Bastion"
+ },
+ {
+ "name": "Gally Bese",
+ "pos_x": 128.78125,
+ "pos_y": -44.1875,
+ "pos_z": 0.5625,
+ "stations": "Nagata Vision"
+ },
+ {
+ "name": "Galonto",
+ "pos_x": 43.46875,
+ "pos_y": -183.25,
+ "pos_z": 82.28125,
+ "stations": "Dyr Platform,Lippisch Dock,Clayton Depot"
+ },
+ {
+ "name": "Galouri",
+ "pos_x": 65.03125,
+ "pos_y": -118.5,
+ "pos_z": 62.5,
+ "stations": "Bahcall Horizons,Argelander Keep,McKean Holdings"
+ },
+ {
+ "name": "Galti",
+ "pos_x": 25.25,
+ "pos_y": -71.25,
+ "pos_z": -61.6875,
+ "stations": "Georg Bothe Gateway,McNair Dock,Lawson Dock,Carr Holdings,Bradshaw Settlement"
+ },
+ {
+ "name": "Gama",
+ "pos_x": -21.625,
+ "pos_y": 26.78125,
+ "pos_z": 127.625,
+ "stations": "Gauss Mine,Sommerfeld Outpost"
+ },
+ {
+ "name": "Gamahine",
+ "pos_x": 161.28125,
+ "pos_y": 11.71875,
+ "pos_z": -3.46875,
+ "stations": "Arber Landing,Ponce de Leon Depot,Dana's Inheritance"
+ },
+ {
+ "name": "Gamamonga",
+ "pos_x": -111.65625,
+ "pos_y": -127.46875,
+ "pos_z": 56.4375,
+ "stations": "Clayton Freeport"
+ },
+ {
+ "name": "Gaman",
+ "pos_x": 114.84375,
+ "pos_y": -120.78125,
+ "pos_z": 36.65625,
+ "stations": "Wheeler Settlement,Bode's Progress,Denning Hub"
+ },
+ {
+ "name": "Gamasakandi",
+ "pos_x": -82.125,
+ "pos_y": 18.40625,
+ "pos_z": -80.3125,
+ "stations": "Ellis Station"
+ },
+ {
+ "name": "Gamatcho",
+ "pos_x": -106.71875,
+ "pos_y": -6.5,
+ "pos_z": -83.125,
+ "stations": "Fabian Hub,Marusek Prospect,Baird Port,Tsunenaga Prospect,Beliaev Legacy"
+ },
+ {
+ "name": "Gambara",
+ "pos_x": 85.1875,
+ "pos_y": -7.75,
+ "pos_z": -72.40625,
+ "stations": "Tarelkin Ring,Haller Holdings,Qureshi Gateway,Chadwick Orbital"
+ },
+ {
+ "name": "Gambiu",
+ "pos_x": 112.5625,
+ "pos_y": -92.125,
+ "pos_z": 26.75,
+ "stations": "Jael Survey,Prandtl Port"
+ },
+ {
+ "name": "Gami Musu",
+ "pos_x": 8.3125,
+ "pos_y": -82.875,
+ "pos_z": 20.0625,
+ "stations": "Chernykh Works,Bisnovatyi-Kogan Hub,Payne-Gaposchkin Point"
+ },
+ {
+ "name": "Gamil",
+ "pos_x": 150.875,
+ "pos_y": -84.78125,
+ "pos_z": -58.15625,
+ "stations": "Kraft Colony,Reis Keep"
+ },
+ {
+ "name": "Gamma Apodis",
+ "pos_x": 107.03125,
+ "pos_y": -55.6875,
+ "pos_z": 99.3125,
+ "stations": "Vess Gateway,Thome Terminal,Brooks Silo"
+ },
+ {
+ "name": "Gamma Coronae Austrinae",
+ "pos_x": -0.46875,
+ "pos_y": -18.71875,
+ "pos_z": 53.25,
+ "stations": "Shuttleworth Hub,Surayev Hub,Franke Beacon,Linteris Port"
+ },
+ {
+ "name": "Gamma Doradus",
+ "pos_x": 46.71875,
+ "pos_y": -46.875,
+ "pos_z": -8.59375,
+ "stations": "Wang City,Swanwick Arena"
+ },
+ {
+ "name": "Gamma Horologii",
+ "pos_x": 116.0625,
+ "pos_y": -138.125,
+ "pos_z": 28.4375,
+ "stations": "Zel'dovich Port"
+ },
+ {
+ "name": "Gamma Hydri",
+ "pos_x": 159.5625,
+ "pos_y": -131.5625,
+ "pos_z": 55,
+ "stations": "Kobayashi Outpost"
+ },
+ {
+ "name": "Gamma Indi",
+ "pos_x": 48.4375,
+ "pos_y": -152.8125,
+ "pos_z": 147.78125,
+ "stations": "Narlikar Base"
+ },
+ {
+ "name": "Gamma Leporis",
+ "pos_x": 19.46875,
+ "pos_y": -11.78125,
+ "pos_z": -18.1875,
+ "stations": "Altman Holdings"
+ },
+ {
+ "name": "Gamma Mensae",
+ "pos_x": 83.3125,
+ "pos_y": -52.84375,
+ "pos_z": 26.9375,
+ "stations": "Brahe Enterprise,Koch Installation,Amnuel's Progress"
+ },
+ {
+ "name": "Gamma Phoenicis",
+ "pos_x": 70.5,
+ "pos_y": -222.46875,
+ "pos_z": 11.15625,
+ "stations": "Unsold Vision,Gamow Relay,Hogg Dock,Barcelona Hub,McQuarrie Survey,Schroeder Prospect,Luyten Orbital,Yourkevitch Installation"
+ },
+ {
+ "name": "Gamma-1 Volantis",
+ "pos_x": 144.78125,
+ "pos_y": -66.15625,
+ "pos_z": 29.78125,
+ "stations": "Kobayashi Base"
+ },
+ {
+ "name": "Gamma-2 Volantis",
+ "pos_x": 126.65625,
+ "pos_y": -57.875,
+ "pos_z": 26.0625,
+ "stations": "Zander Landing,Tem Lab,Emshwiller Terminal"
+ },
+ {
+ "name": "Gamma-3 Octantis",
+ "pos_x": 171.53125,
+ "pos_y": -146.25,
+ "pos_z": 118.34375,
+ "stations": "Bruck Platform,Pu Point"
+ },
+ {
+ "name": "Gan",
+ "pos_x": -41,
+ "pos_y": -90.34375,
+ "pos_z": 30.875,
+ "stations": "Zelazny Enterprise,Verrazzano City,Moore Enterprise"
+ },
+ {
+ "name": "Ganda",
+ "pos_x": 25.40625,
+ "pos_y": -22.5,
+ "pos_z": -104.4375,
+ "stations": "Shepard's Claim,Collins Mines"
+ },
+ {
+ "name": "Gandagetu",
+ "pos_x": 65.25,
+ "pos_y": 62.40625,
+ "pos_z": 129.8125,
+ "stations": "Leinster Holdings,Scithers Orbital,Ackerman Enterprise,Rothfuss Plant"
+ },
+ {
+ "name": "Gandha Wu",
+ "pos_x": 38.25,
+ "pos_y": -198.5,
+ "pos_z": 81.34375,
+ "stations": "Burnham Port,Bahcall Vision,Beekman Lab,Chadwick Station,Rodrigues Base"
+ },
+ {
+ "name": "Gandharvi",
+ "pos_x": -6703.5,
+ "pos_y": -157.84375,
+ "pos_z": 14108.03125,
+ "stations": "Caravanserai,Marlin's Reach"
+ },
+ {
+ "name": "Gandhs",
+ "pos_x": 45.15625,
+ "pos_y": -45.34375,
+ "pos_z": 108.78125,
+ "stations": "Sucharitkul's Progress,Ellis Dock,Ciferri Mines"
+ },
+ {
+ "name": "Gandigi",
+ "pos_x": -19.65625,
+ "pos_y": 12,
+ "pos_z": 144.59375,
+ "stations": "Ryumin City"
+ },
+ {
+ "name": "Gandii",
+ "pos_x": -84.4375,
+ "pos_y": -102.5,
+ "pos_z": 33.25,
+ "stations": "Lu Hub,Carver's Claim,Babbage Station,Erikson Forum,Gorbatko Enterprise"
+ },
+ {
+ "name": "Gandillava",
+ "pos_x": 92.125,
+ "pos_y": -123.21875,
+ "pos_z": 132.78125,
+ "stations": "Jones Horizons,Miller City,Bennett Dock,Taylor Landing,Vess Survey"
+ },
+ {
+ "name": "Gandin",
+ "pos_x": 36.5625,
+ "pos_y": 80.59375,
+ "pos_z": -139.375,
+ "stations": "Hassanein Plant"
+ },
+ {
+ "name": "Gandje",
+ "pos_x": 79.78125,
+ "pos_y": -66.875,
+ "pos_z": -131.0625,
+ "stations": "Williamson Ring"
+ },
+ {
+ "name": "Gandjeraiti",
+ "pos_x": -40.125,
+ "pos_y": -159.28125,
+ "pos_z": -60.4375,
+ "stations": "Pearse Mines"
+ },
+ {
+ "name": "Gandones",
+ "pos_x": -18.1875,
+ "pos_y": -29.96875,
+ "pos_z": 116.90625,
+ "stations": "Yegorov Terminal,Hawley Station,Bain Ring,Smith Orbital,Furukawa Enterprise,al-Din Dock,Murray Terminal,Navigator Beacon,Jones Penal colony"
+ },
+ {
+ "name": "Gandoques",
+ "pos_x": 118.59375,
+ "pos_y": -215.90625,
+ "pos_z": 86.84375,
+ "stations": "Sweet Works"
+ },
+ {
+ "name": "Gandra",
+ "pos_x": 48.46875,
+ "pos_y": -85.75,
+ "pos_z": -45.3125,
+ "stations": "May Camp"
+ },
+ {
+ "name": "Gandu",
+ "pos_x": -69.6875,
+ "pos_y": -87.84375,
+ "pos_z": -112.59375,
+ "stations": "Swigert Terminal,Pogue Port,Ramanujan Installation"
+ },
+ {
+ "name": "Gandui",
+ "pos_x": -18.375,
+ "pos_y": -153.46875,
+ "pos_z": 48.8125,
+ "stations": "Regiomontanus Ring,Kuttner Settlement,Dixon Dock,Lopez-Garcia Gateway"
+ },
+ {
+ "name": "Gandvik",
+ "pos_x": 55.21875,
+ "pos_y": 3.9375,
+ "pos_z": -85.625,
+ "stations": "Chorel Port,Pullman Base,Ordway Settlement"
+ },
+ {
+ "name": "Gandza",
+ "pos_x": 102.9375,
+ "pos_y": -15.34375,
+ "pos_z": -63.3125,
+ "stations": "Tesla Dock,Rominger Ring"
+ },
+ {
+ "name": "Ganebucae",
+ "pos_x": 139.6875,
+ "pos_y": -45,
+ "pos_z": 26.21875,
+ "stations": "Beg Horizons,Saarinen Station,Wilhelm Bastion,Banno Hub"
+ },
+ {
+ "name": "Ganekpe",
+ "pos_x": -88.3125,
+ "pos_y": -43.84375,
+ "pos_z": 160.84375,
+ "stations": "McMullen Hub,Kier Gateway,Pangborn Port,Nicholson Keep"
+ },
+ {
+ "name": "Gang Yun",
+ "pos_x": 29.8125,
+ "pos_y": 140.71875,
+ "pos_z": 34.75,
+ "stations": "Appel Outpost,Mackenzie Installation"
+ },
+ {
+ "name": "Gangbetae",
+ "pos_x": -103.625,
+ "pos_y": -31.21875,
+ "pos_z": 92.6875,
+ "stations": "Williams Reformatory"
+ },
+ {
+ "name": "Gangga",
+ "pos_x": 113.3125,
+ "pos_y": -123.4375,
+ "pos_z": 149.125,
+ "stations": "Wigura Station,Curtis Dock,Barbuy Barracks,Clark Station,Fisk Landing"
+ },
+ {
+ "name": "Gango",
+ "pos_x": 124.46875,
+ "pos_y": 2.625,
+ "pos_z": 21.75,
+ "stations": "Cousin Keep,Archambault Plant,Euclid Platform"
+ },
+ {
+ "name": "Gangu",
+ "pos_x": 110.875,
+ "pos_y": 44.78125,
+ "pos_z": -28.375,
+ "stations": "McQuay Colony,Atwood Orbital"
+ },
+ {
+ "name": "Gani",
+ "pos_x": 59.21875,
+ "pos_y": -13.8125,
+ "pos_z": -79.25,
+ "stations": "Williams Ring,Rashid Depot"
+ },
+ {
+ "name": "Ganiklis",
+ "pos_x": 23.40625,
+ "pos_y": -6.4375,
+ "pos_z": -38.9375,
+ "stations": "Willis Vision,Piper Platform"
+ },
+ {
+ "name": "Ganit",
+ "pos_x": 54.875,
+ "pos_y": -131.40625,
+ "pos_z": -63.9375,
+ "stations": "Mooz Platform,Hirasawa Depot"
+ },
+ {
+ "name": "Ganiyan",
+ "pos_x": 88.71875,
+ "pos_y": 87.4375,
+ "pos_z": 121.21875,
+ "stations": "Erikson Dock,Bretnor Port,Crowley Works,Herreshoff Terminal,Koontz Installation"
+ },
+ {
+ "name": "Gaoh",
+ "pos_x": -65.625,
+ "pos_y": 108.0625,
+ "pos_z": 104.1875,
+ "stations": "Hawking Station,Nusslein-Volhard Enterprise,Hardwick Platform,Kapp Holdings"
+ },
+ {
+ "name": "Gaohikel",
+ "pos_x": 49.53125,
+ "pos_y": 33.28125,
+ "pos_z": 57.125,
+ "stations": "Dalton Dock,Behnken Settlement,Wittgenstein Port,Lopez de Legazpi Base"
+ },
+ {
+ "name": "Gaohim",
+ "pos_x": -6.15625,
+ "pos_y": 116.1875,
+ "pos_z": 95,
+ "stations": "Abasheli Terminal,Galois Hub,Dukaj Point"
+ },
+ {
+ "name": "Gaohima",
+ "pos_x": 156.59375,
+ "pos_y": -66.46875,
+ "pos_z": 50.625,
+ "stations": "Ziegler Colony"
+ },
+ {
+ "name": "Gaoshe",
+ "pos_x": -137.46875,
+ "pos_y": -6.15625,
+ "pos_z": 37.6875,
+ "stations": "Tilman Horizons,Potrykus Horizons,Carrier Plant"
+ },
+ {
+ "name": "Garabaei",
+ "pos_x": -40.59375,
+ "pos_y": -102.625,
+ "pos_z": 68.90625,
+ "stations": "Ampere Hub,Laval Hub,Tito Terminal,Killough Plant,Ballard Relay"
+ },
+ {
+ "name": "Garama",
+ "pos_x": -143,
+ "pos_y": 57.3125,
+ "pos_z": 4.96875,
+ "stations": "Maire Refinery,Berners-Lee Terminal,Dana Dock"
+ },
+ {
+ "name": "Garanji",
+ "pos_x": 154.90625,
+ "pos_y": 29.8125,
+ "pos_z": -25.3125,
+ "stations": "Volta Point,Kelly Prospect,Prunariu Colony"
+ },
+ {
+ "name": "Garib",
+ "pos_x": -60.28125,
+ "pos_y": 30.03125,
+ "pos_z": -71.75,
+ "stations": "Caillie Settlement,Whitney City,Junlong Platform"
+ },
+ {
+ "name": "Garinanni",
+ "pos_x": -74.8125,
+ "pos_y": -138.6875,
+ "pos_z": 1.9375,
+ "stations": "Andersson Station,Vonarburg City,Bernoulli Penal colony"
+ },
+ {
+ "name": "Garitio",
+ "pos_x": 79.0625,
+ "pos_y": -62.6875,
+ "pos_z": -43.9375,
+ "stations": "Lindstrand Settlement,Hevelius Port,Kanai Asylum"
+ },
+ {
+ "name": "Garm",
+ "pos_x": 50.6875,
+ "pos_y": 15.125,
+ "pos_z": 138.125,
+ "stations": "Chilton Port,Vernadsky Settlement,Wallis Laboratory"
+ },
+ {
+ "name": "Garma",
+ "pos_x": 78.09375,
+ "pos_y": -241.8125,
+ "pos_z": 62.375,
+ "stations": "Kimura Settlement"
+ },
+ {
+ "name": "Garoju",
+ "pos_x": 122.03125,
+ "pos_y": -126.1875,
+ "pos_z": 120.09375,
+ "stations": "Verrier Orbital,Galle Orbital,Kapteyn Dock,Puleston Beacon"
+ },
+ {
+ "name": "Garoku",
+ "pos_x": -99.75,
+ "pos_y": -58,
+ "pos_z": -61.15625,
+ "stations": "West Port,Tevis Terminal,Griffin Hub,Toll Station,Terry Port,Stackpole Terminal,Mitchell Orbital,Meredith's Pride,de Caminha Point,Meaney Barracks"
+ },
+ {
+ "name": "Garongxians",
+ "pos_x": -43.9375,
+ "pos_y": -131.9375,
+ "pos_z": -94.84375,
+ "stations": "Vries Terminal,Xuanzang Vision,Schmitz Survey,Kier Depot,Haldeman Terminal"
+ },
+ {
+ "name": "Garrinbatz",
+ "pos_x": 32.75,
+ "pos_y": -223.09375,
+ "pos_z": 64.28125,
+ "stations": "Common Enterprise,Neugebauer Hub,Lewis Port,Timofeyevich Vision,Tiptree Beacon"
+ },
+ {
+ "name": "Garuda",
+ "pos_x": -9553.46875,
+ "pos_y": -914.75,
+ "pos_z": 19812.28125,
+ "stations": "Vera Rubin Complex"
+ },
+ {
+ "name": "Garudhumir",
+ "pos_x": 45.6875,
+ "pos_y": 1.4375,
+ "pos_z": 159.40625,
+ "stations": "Weil Terminal"
+ },
+ {
+ "name": "Garuna",
+ "pos_x": 14.625,
+ "pos_y": -23.9375,
+ "pos_z": 85.15625,
+ "stations": "Otiman Prospect,Yegorov Port,Banks Silo"
+ },
+ {
+ "name": "Garup",
+ "pos_x": -21.75,
+ "pos_y": -33.4375,
+ "pos_z": -93.0625,
+ "stations": "Citi Port"
+ },
+ {
+ "name": "Garuttii",
+ "pos_x": 38.90625,
+ "pos_y": 28.21875,
+ "pos_z": 131.625,
+ "stations": "Song Vision,Ramelli Terminal,Caselberg Prospect"
+ },
+ {
+ "name": "Garuwa",
+ "pos_x": -130.6875,
+ "pos_y": -51.3125,
+ "pos_z": -74.46875,
+ "stations": "Thesiger Landing,Csoma Sanctuary,Cook Enterprise"
+ },
+ {
+ "name": "GAT 2",
+ "pos_x": -35.3125,
+ "pos_y": 17.71875,
+ "pos_z": 58.875,
+ "stations": "Thompson Vision"
+ },
+ {
+ "name": "Gateway",
+ "pos_x": -11,
+ "pos_y": 77.84375,
+ "pos_z": -0.875,
+ "stations": "Dublin Citadel,Wicca Town,New Chernobyl,Barbosa Lab,Kirtley Dock,Mackenzie Hub,Fancher Dock,Blish Survey"
+ },
+ {
+ "name": "Gato",
+ "pos_x": -69.5625,
+ "pos_y": -0.03125,
+ "pos_z": 9.21875,
+ "stations": "Brongniart Ring,Hoffman Penal colony,Potrykus Market,Stebler Landing,Harper Survey"
+ },
+ {
+ "name": "Gatoborod",
+ "pos_x": -20.90625,
+ "pos_y": -12.09375,
+ "pos_z": 152.625,
+ "stations": "Roberts Enterprise,Selye Port,Carr Vision,Williams Refinery"
+ },
+ {
+ "name": "Gatolla",
+ "pos_x": -102.46875,
+ "pos_y": -55.34375,
+ "pos_z": 96.25,
+ "stations": "Tanner Orbital,Malaspina Holdings,Virts Gateway,Eratosthenes Gateway,Fernandes Settlement,Haignere Vision"
+ },
+ {
+ "name": "Gatondos",
+ "pos_x": 98.34375,
+ "pos_y": -20.9375,
+ "pos_z": 102,
+ "stations": "Heisenberg Settlement,Reed Enterprise"
+ },
+ {
+ "name": "Gatonese",
+ "pos_x": 33.40625,
+ "pos_y": 19.53125,
+ "pos_z": -92.3125,
+ "stations": "Shaver's Claim,Simak Keep,Darlton's Progress"
+ },
+ {
+ "name": "Gatorma",
+ "pos_x": -80.5,
+ "pos_y": -92.90625,
+ "pos_z": 64.8125,
+ "stations": "Williams Horizons"
+ },
+ {
+ "name": "Gaudu",
+ "pos_x": 153.46875,
+ "pos_y": -108.78125,
+ "pos_z": -3.34375,
+ "stations": "Airy Relay,Giclas Base"
+ },
+ {
+ "name": "Gaula",
+ "pos_x": 90.625,
+ "pos_y": -2.65625,
+ "pos_z": 76.90625,
+ "stations": "McQuay Port,Ledyard Terminal,J. G. Ballard Station,MacVicar Point,Hahn Barracks,Mallory Observatory,Gallun Terminal,Hiraga Hub,Stillman Port,Shiner Laboratory,Pashin's Claim"
+ },
+ {
+ "name": "Gaula Saon",
+ "pos_x": -103.03125,
+ "pos_y": -30.21875,
+ "pos_z": -107.0625,
+ "stations": "Atwater Orbital"
+ },
+ {
+ "name": "Gaula Wu",
+ "pos_x": 90.03125,
+ "pos_y": -96.1875,
+ "pos_z": 5.28125,
+ "stations": "Cowling Dock,Friedman Works,Puiseux Orbital,Sy Laboratory,Wenzel Hub,Bellamy Refinery"
+ },
+ {
+ "name": "Gauluujja",
+ "pos_x": -60.75,
+ "pos_y": -142,
+ "pos_z": -43.53125,
+ "stations": "Weitz Ring,Martinez Holdings,Armstrong Gateway,Adams Port,Hoffman Settlement"
+ },
+ {
+ "name": "Gaunab",
+ "pos_x": 1.8125,
+ "pos_y": -108.28125,
+ "pos_z": -22.90625,
+ "stations": "Veron Port,Unsold Settlement,Giacconi Platform,Ziewe Installation,Martiniere's Inheritance"
+ },
+ {
+ "name": "Gaunetet",
+ "pos_x": 81.6875,
+ "pos_y": -255.75,
+ "pos_z": 102.6875,
+ "stations": "Fischer Orbital,Artsutanov Prospect,Pilcher Penal colony"
+ },
+ {
+ "name": "Gaura",
+ "pos_x": -82.40625,
+ "pos_y": -63.84375,
+ "pos_z": -1.65625,
+ "stations": "Rocklynne Base,Ahern Dock,Blackwell Vision,Norman Terminal,Galois Orbital,Brust Bastion"
+ },
+ {
+ "name": "Gautama",
+ "pos_x": -6.78125,
+ "pos_y": -90.21875,
+ "pos_z": -17.8125,
+ "stations": "Blaylock Dock,Kennicott Holdings"
+ },
+ {
+ "name": "Gautas",
+ "pos_x": -82.96875,
+ "pos_y": 64.21875,
+ "pos_z": -92.53125,
+ "stations": "Levy Dock,Hoshi Enterprise,Mourelle Port,Bates Vision"
+ },
+ {
+ "name": "Gauts",
+ "pos_x": 138.96875,
+ "pos_y": -55.21875,
+ "pos_z": 39,
+ "stations": "Angstrom Prospect,Argelander Mines,Kress Refinery,Gabriel Keep"
+ },
+ {
+ "name": "Gbekree",
+ "pos_x": 78.5,
+ "pos_y": -46.59375,
+ "pos_z": -38.6875,
+ "stations": "Dzhanibekov Port,Kendrick Plant,Ferguson Relay,Hurley Dock"
+ },
+ {
+ "name": "Gciri",
+ "pos_x": 136.96875,
+ "pos_y": -124.15625,
+ "pos_z": 57.90625,
+ "stations": "Quetelet Outpost"
+ },
+ {
+ "name": "Gciriko",
+ "pos_x": 3,
+ "pos_y": -103.84375,
+ "pos_z": -131.9375,
+ "stations": "Iqbal Installation,Kroehl Landing,d'Eyncourt Landing"
+ },
+ {
+ "name": "Gcirisci",
+ "pos_x": -108.5,
+ "pos_y": 52.65625,
+ "pos_z": 88.03125,
+ "stations": "Nobel Port"
+ },
+ {
+ "name": "Gcirithang",
+ "pos_x": 63.4375,
+ "pos_y": -34.25,
+ "pos_z": 24.1875,
+ "stations": "Bykovsky Terminal"
+ },
+ {
+ "name": "Gcirthi",
+ "pos_x": -63.34375,
+ "pos_y": -76.28125,
+ "pos_z": 53.5,
+ "stations": "Kent Station,Isherwood Dock,Vizcaino Depot,Steakley Port"
+ },
+ {
+ "name": "GCRV 13292",
+ "pos_x": -99.84375,
+ "pos_y": 27.5625,
+ "pos_z": -28.1875,
+ "stations": "Noriega Installation,Walheim Dock"
+ },
+ {
+ "name": "GCRV 1526",
+ "pos_x": -70.125,
+ "pos_y": -18.875,
+ "pos_z": -88.21875,
+ "stations": "Melroy's Claim"
+ },
+ {
+ "name": "GCRV 1568",
+ "pos_x": -33.90625,
+ "pos_y": -63,
+ "pos_z": -82.875,
+ "stations": "Cernan Dock,Humboldt City,Gagnan Hub,Kube-McDowell Base,Milnor Survey,Chomsky Terminal,Clerk Hub"
+ },
+ {
+ "name": "GCRV 2311",
+ "pos_x": -37.375,
+ "pos_y": -16.75,
+ "pos_z": -97.3125,
+ "stations": "Dunn Mines,Kanwar Settlement"
+ },
+ {
+ "name": "GCRV 2743",
+ "pos_x": -69.375,
+ "pos_y": 28.59375,
+ "pos_z": -93.03125,
+ "stations": "Cormack Dock,Velho Point,Noakes Market,Chiao City,Readdy Hub,Broglie Holdings,Krylov Palace,Carson Port"
+ },
+ {
+ "name": "GCRV 3034",
+ "pos_x": -4.34375,
+ "pos_y": -16.09375,
+ "pos_z": -117.53125,
+ "stations": "Foreman Orbital,Gottlob Frege Laboratory"
+ },
+ {
+ "name": "GCRV 3204",
+ "pos_x": 62.625,
+ "pos_y": -51.15625,
+ "pos_z": -85.59375,
+ "stations": "Donaldson Gateway,Carstensz Ring,Veblen Ring"
+ },
+ {
+ "name": "GCRV 376",
+ "pos_x": -26.46875,
+ "pos_y": -114.625,
+ "pos_z": -12.625,
+ "stations": "Tarski Station,Ing Hub"
+ },
+ {
+ "name": "GCRV 3791",
+ "pos_x": -58.84375,
+ "pos_y": 41.03125,
+ "pos_z": -83.40625,
+ "stations": "Coleman Hub,Meikle Gateway,Brady Installation"
+ },
+ {
+ "name": "GCRV 4259",
+ "pos_x": 13.3125,
+ "pos_y": 18.09375,
+ "pos_z": -105.65625,
+ "stations": "Puleston City,Veblen Point"
+ },
+ {
+ "name": "GCRV 4654",
+ "pos_x": 12.78125,
+ "pos_y": 21.125,
+ "pos_z": -74.375,
+ "stations": "Asire Base,Herzfeld Landing"
+ },
+ {
+ "name": "GCRV 52424",
+ "pos_x": -7.90625,
+ "pos_y": -98.28125,
+ "pos_z": -42,
+ "stations": "Kadenyuk Orbital,Karl Diesel Orbital,Carey Enterprise,Artyukhin Port,Meade Ring,Thomson Port,Spring Ring,Holden Horizons,Kozeyev Enterprise,Williams Settlement,Bombelli Prospect,Cogswell Keep"
+ },
+ {
+ "name": "GCRV 54903",
+ "pos_x": -11.75,
+ "pos_y": -34.875,
+ "pos_z": -100.21875,
+ "stations": "Morgan Freeport"
+ },
+ {
+ "name": "GCRV 60778",
+ "pos_x": 34.3125,
+ "pos_y": 76.40625,
+ "pos_z": -63.375,
+ "stations": "Wiley Terminal,Wilcutt Port"
+ },
+ {
+ "name": "GCRV 60796",
+ "pos_x": 111.4375,
+ "pos_y": 3.375,
+ "pos_z": 8.75,
+ "stations": "Burroughs Camp,MacVicar Colony"
+ },
+ {
+ "name": "GCRV 61007",
+ "pos_x": 103.90625,
+ "pos_y": 20.03125,
+ "pos_z": 2.0625,
+ "stations": "Berners-Lee Orbital,Ohm Dock,Bobko Station"
+ },
+ {
+ "name": "GCRV 61377",
+ "pos_x": 31.03125,
+ "pos_y": 99.5,
+ "pos_z": -51.0625,
+ "stations": "Godwin Hub,Mallett Port,Rolland Hub"
+ },
+ {
+ "name": "GCRV 62586",
+ "pos_x": 30.78125,
+ "pos_y": 97.6875,
+ "pos_z": -17.125,
+ "stations": "Ing Station,Piercy Installation,Markov Hub,Peary Hangar"
+ },
+ {
+ "name": "GCRV 6406",
+ "pos_x": -54.25,
+ "pos_y": 80.71875,
+ "pos_z": -68.875,
+ "stations": "Coulter Dock"
+ },
+ {
+ "name": "GCRV 65163",
+ "pos_x": -55.6875,
+ "pos_y": 93.28125,
+ "pos_z": 13.1875,
+ "stations": "Hovgaard Dock"
+ },
+ {
+ "name": "GCRV 65988",
+ "pos_x": -78.21875,
+ "pos_y": 86.5625,
+ "pos_z": 21.8125,
+ "stations": "Treshchov Hub,Bendell Horizons"
+ },
+ {
+ "name": "GCRV 7300",
+ "pos_x": -36.78125,
+ "pos_y": 103.0625,
+ "pos_z": -37.28125,
+ "stations": "Butler Colony,Pribylov Vision,Eschbach Enterprise,Bartlett Hub"
+ },
+ {
+ "name": "GD 1192",
+ "pos_x": -22.28125,
+ "pos_y": -67.84375,
+ "pos_z": 12.5,
+ "stations": "Melvin Hub,Kotov City,Wheeler Arsenal"
+ },
+ {
+ "name": "GD 140",
+ "pos_x": 4.84375,
+ "pos_y": 49.5625,
+ "pos_z": -13.3125,
+ "stations": "Liwei Terminal,Newton Hub,Levi-Montalcini Port,Vaugh Installation,Bosch Beacon,Viehbock Dock,Anning Orbital,Bosch Enterprise"
+ },
+ {
+ "name": "GD 175",
+ "pos_x": 9.3125,
+ "pos_y": 56.53125,
+ "pos_z": 60.59375,
+ "stations": "Wegener Terminal,Rutherford Ring,Wellman Port,Hopper Penal colony,Champlain Base"
+ },
+ {
+ "name": "GD 215",
+ "pos_x": -47.25,
+ "pos_y": 5.28125,
+ "pos_z": 65.25,
+ "stations": "Kingsbury Base,Faraday Orbital"
+ },
+ {
+ "name": "GD 219",
+ "pos_x": -48.65625,
+ "pos_y": 0.125,
+ "pos_z": 41.6875,
+ "stations": "McKee Ring"
+ },
+ {
+ "name": "GD 279",
+ "pos_x": -35.25,
+ "pos_y": -12.46875,
+ "pos_z": -34.0625,
+ "stations": "Sinclair Gateway,Camarda Orbital,Zalyotin Survey,Volynov Enterprise"
+ },
+ {
+ "name": "GD 319",
+ "pos_x": -19.375,
+ "pos_y": 43.625,
+ "pos_z": -12.75,
+ "stations": "Bose Landing,Pontes Point,Melnick Settlement,Bhabha Terminal"
+ },
+ {
+ "name": "GD 69",
+ "pos_x": -12.4375,
+ "pos_y": 5.375,
+ "pos_z": -60.0625,
+ "stations": "Nikolayev Stop"
+ },
+ {
+ "name": "Ge",
+ "pos_x": 82.40625,
+ "pos_y": -50.9375,
+ "pos_z": -134.46875,
+ "stations": "MacLeod Dock,Evangelisti Enterprise,Sharp Dock,Riemann Dock"
+ },
+ {
+ "name": "Geawen",
+ "pos_x": 22.5,
+ "pos_y": 23.78125,
+ "pos_z": 171.0625,
+ "stations": "Obruchev Legacy,Wnuk-Lipinski Landing,Rond d'Alembert Legacy"
+ },
+ {
+ "name": "Geawenki",
+ "pos_x": 11.59375,
+ "pos_y": 15.625,
+ "pos_z": -71.625,
+ "stations": "Knapp Vision,Kozin Vision"
+ },
+ {
+ "name": "Geawer",
+ "pos_x": 55.6875,
+ "pos_y": -138.375,
+ "pos_z": 129.4375,
+ "stations": "Roe Station,Dalgarno Hub,Aitken Keep"
+ },
+ {
+ "name": "Geawete",
+ "pos_x": 39.125,
+ "pos_y": 6.96875,
+ "pos_z": 55.53125,
+ "stations": "Fontana Enterprise,Currie Dock,Great's Pride,Brust Port,Sevastyanov Dock"
+ },
+ {
+ "name": "Geawetes",
+ "pos_x": -2.25,
+ "pos_y": -214.5,
+ "pos_z": -15.40625,
+ "stations": "Bartini City,Hirayama Dock,Elson Settlement,Bergerac Bastion,Saberhagen Refinery"
+ },
+ {
+ "name": "Geb",
+ "pos_x": 16.125,
+ "pos_y": 38.8125,
+ "pos_z": 60,
+ "stations": "Bryusov Orbital,Pordenone Terminal,Ellison Dock,Eckford Depot"
+ },
+ {
+ "name": "Gebel",
+ "pos_x": 23.40625,
+ "pos_y": -50.90625,
+ "pos_z": 29.375,
+ "stations": "Michell Port,Schoening City,Soper Beacon,van de Sande Bakhuyzen Station,Riess Hub,Richer Vision,Robert Dock,Struve's Progress,Xin Port,Youll Hub,Theodorsen Station,Bushkov Settlement,Pournelle Installation,Newman Colony,Carlisle Depot"
+ },
+ {
+ "name": "Gefion",
+ "pos_x": 107.53125,
+ "pos_y": 48.6875,
+ "pos_z": 107.3125,
+ "stations": "Poisson Mines,Narbeth Survey"
+ },
+ {
+ "name": "Gefjong",
+ "pos_x": 103.53125,
+ "pos_y": -183.40625,
+ "pos_z": 26.21875,
+ "stations": "Maughmer Base,Baliunas Hub"
+ },
+ {
+ "name": "Gefjotec",
+ "pos_x": 37.46875,
+ "pos_y": -78.78125,
+ "pos_z": -90.9375,
+ "stations": "Atkov Platform,Molina Terminal"
+ },
+ {
+ "name": "Geidjaramo",
+ "pos_x": 4.875,
+ "pos_y": 54.84375,
+ "pos_z": 141.875,
+ "stations": "Rzeppa City,Gauss Platform,Akers Installation"
+ },
+ {
+ "name": "Geidmara",
+ "pos_x": 51.78125,
+ "pos_y": -82.125,
+ "pos_z": -49.125,
+ "stations": "Rosenberg Survey,Ferguson Dock,Harawi Hub,Potez Station"
+ },
+ {
+ "name": "Geidubiabos",
+ "pos_x": -36,
+ "pos_y": -119.15625,
+ "pos_z": -76.03125,
+ "stations": "Blaha Orbital,Ross Refinery"
+ },
+ {
+ "name": "Geirobri",
+ "pos_x": 148.46875,
+ "pos_y": -117.125,
+ "pos_z": 21.5625,
+ "stations": "Addams Ring"
+ },
+ {
+ "name": "Geirok",
+ "pos_x": 140.71875,
+ "pos_y": -80.75,
+ "pos_z": 33.21875,
+ "stations": "Ghez Dock,Roche Colony"
+ },
+ {
+ "name": "Geirsemi",
+ "pos_x": 65.5,
+ "pos_y": -114,
+ "pos_z": -66.09375,
+ "stations": "Palitzsch Orbital,Gurevich Station,Schaumasse Colony,Filippenko Observatory,Neville Base"
+ },
+ {
+ "name": "Gelo Kop",
+ "pos_x": 108.21875,
+ "pos_y": -111.25,
+ "pos_z": 124.46875,
+ "stations": "August von Steinheil Terminal,Pickering Port,Morrill Dock,Shunkai's Progress"
+ },
+ {
+ "name": "Gelong",
+ "pos_x": -3.90625,
+ "pos_y": -83.0625,
+ "pos_z": 66.84375,
+ "stations": "Roe Ring,Utkin Enterprise,Bryusov Laboratory,Dunlop Hub"
+ },
+ {
+ "name": "Gendalla",
+ "pos_x": 12,
+ "pos_y": 9.96875,
+ "pos_z": -5.53125,
+ "stations": "Aksyonov Installation,Clervoy City,Back Holdings,Ivins City,Garay Port"
+ },
+ {
+ "name": "Gende",
+ "pos_x": 93.28125,
+ "pos_y": -117.75,
+ "pos_z": -1.46875,
+ "stations": "Baliunas Hub,Ocampo Enterprise,Albitzky Keep"
+ },
+ {
+ "name": "Gendens",
+ "pos_x": 68.46875,
+ "pos_y": -203.5,
+ "pos_z": -14.25,
+ "stations": "Oren Station,Brill Station,Tietjen Vision,Arai Refinery,Barbosa Installation"
+ },
+ {
+ "name": "Gendenwitha",
+ "pos_x": -116.28125,
+ "pos_y": -6.28125,
+ "pos_z": 115.34375,
+ "stations": "Filipchenko Gateway,Herrington Gateway,Kanwar Terminal,Scortia Camp"
+ },
+ {
+ "name": "Gender",
+ "pos_x": 59,
+ "pos_y": -124.6875,
+ "pos_z": 7.84375,
+ "stations": "Dhawan Survey,Moore Hub,Maanen Laboratory"
+ },
+ {
+ "name": "Genderi",
+ "pos_x": -50.875,
+ "pos_y": 15.15625,
+ "pos_z": 119.5625,
+ "stations": "Wallace Point,Spielberg Installation,Malzberg Terminal,Manning Port,Sargent Terminal,Kennicott Orbital,Webb Orbital"
+ },
+ {
+ "name": "Gendi",
+ "pos_x": 152.46875,
+ "pos_y": -177.9375,
+ "pos_z": 80.15625,
+ "stations": "Boyajian's Claim"
+ },
+ {
+ "name": "Gendini",
+ "pos_x": -122.5625,
+ "pos_y": 48.5,
+ "pos_z": -34.5625,
+ "stations": "Spring Prospect,Chu Hub,Bell Terminal,Seddon Observatory"
+ },
+ {
+ "name": "George Pantazis",
+ "pos_x": -12.09375,
+ "pos_y": -16,
+ "pos_z": -14.21875,
+ "stations": "Schmitt's Progress,Zamka Platform,Melvin Hangar,Banks Refinery,Crampton's Claim"
+ },
+ {
+ "name": "Gera",
+ "pos_x": -88.8125,
+ "pos_y": 11.6875,
+ "pos_z": -33.59375,
+ "stations": "Stebler Hub,Aksyonov Dock"
+ },
+ {
+ "name": "Geras",
+ "pos_x": -7.03125,
+ "pos_y": -12.875,
+ "pos_z": -56.375,
+ "stations": "Yurchikhin Port,Kondratyev Port,Noakes Holdings,Lehtonen Terminal,Sadi Carnot Dock"
+ },
+ {
+ "name": "Gerdan",
+ "pos_x": 116.625,
+ "pos_y": -53.78125,
+ "pos_z": -120.46875,
+ "stations": "Grego Freeport,McMullen's Progress"
+ },
+ {
+ "name": "Gerdhr",
+ "pos_x": -73.21875,
+ "pos_y": 162.5,
+ "pos_z": -22.625,
+ "stations": "Laveykin Reach,Auld Hangar,Young Terminal"
+ },
+ {
+ "name": "Gerdia",
+ "pos_x": 202.09375,
+ "pos_y": -182.46875,
+ "pos_z": -2.78125,
+ "stations": "Hollander Dock,Ahmed Keep"
+ },
+ {
+ "name": "Gerdisao",
+ "pos_x": -110.53125,
+ "pos_y": -105.625,
+ "pos_z": 37.125,
+ "stations": "Lee Settlement,Selberg Survey,Rangarajan Orbital"
+ },
+ {
+ "name": "Gerdulla",
+ "pos_x": 63.75,
+ "pos_y": -263.625,
+ "pos_z": 8.125,
+ "stations": "Seyfert Vision,Potocnik Works,Bolton Observatory"
+ },
+ {
+ "name": "Gero Kiaku",
+ "pos_x": 135.90625,
+ "pos_y": -48.375,
+ "pos_z": 4.96875,
+ "stations": "Chiang Port"
+ },
+ {
+ "name": "Geroer",
+ "pos_x": 54.25,
+ "pos_y": -128.5,
+ "pos_z": 70.25,
+ "stations": "Mayer Mines,Donati Bastion,Wilson Hub,Fraknoi Vision"
+ },
+ {
+ "name": "Gerong",
+ "pos_x": 88.53125,
+ "pos_y": 10.5,
+ "pos_z": -142.09375,
+ "stations": "Ciferri Orbital,Arnold Settlement,Sheffield Prospect"
+ },
+ {
+ "name": "Gers",
+ "pos_x": 151.5,
+ "pos_y": 10.84375,
+ "pos_z": 32.84375,
+ "stations": "Jenkinson Orbital"
+ },
+ {
+ "name": "Gerseklis",
+ "pos_x": 126.1875,
+ "pos_y": -66.03125,
+ "pos_z": -8,
+ "stations": "Burgess Relay,Haldeman Installation"
+ },
+ {
+ "name": "Gerthel",
+ "pos_x": 130.28125,
+ "pos_y": -20.8125,
+ "pos_z": -2.4375,
+ "stations": "Boulton Port"
+ },
+ {
+ "name": "Gertrud",
+ "pos_x": -13.28125,
+ "pos_y": 6.90625,
+ "pos_z": -56.59375,
+ "stations": "Walker Ring"
+ },
+ {
+ "name": "Gertsi",
+ "pos_x": 26.6875,
+ "pos_y": 57.03125,
+ "pos_z": 153.15625,
+ "stations": "Malerba Camp"
+ },
+ {
+ "name": "Gertsic",
+ "pos_x": 45.21875,
+ "pos_y": -33.84375,
+ "pos_z": 155.21875,
+ "stations": "Gernhardt Mines,Binnie Point,Tilley Plant"
+ },
+ {
+ "name": "Ghara",
+ "pos_x": -0.84375,
+ "pos_y": 25.71875,
+ "pos_z": 140.375,
+ "stations": "Cavalieri Station"
+ },
+ {
+ "name": "Gharing",
+ "pos_x": -23.90625,
+ "pos_y": -20.8125,
+ "pos_z": 101.5,
+ "stations": "Still Orbital,Skripochka Terminal,Bruce Exchange,Gagnan Orbital"
+ },
+ {
+ "name": "Ghary",
+ "pos_x": 133.15625,
+ "pos_y": 86.9375,
+ "pos_z": -136.625,
+ "stations": "Francisco de Eliza Survey,Shawl Beacon"
+ },
+ {
+ "name": "Ghede",
+ "pos_x": 32.6875,
+ "pos_y": 59.1875,
+ "pos_z": 95.6875,
+ "stations": "Al Saud Vision,Jahn Horizons,Laval Colony"
+ },
+ {
+ "name": "Ghekree",
+ "pos_x": -76.3125,
+ "pos_y": -76.6875,
+ "pos_z": 104.4375,
+ "stations": "Heceta Terminal,Riemann Enterprise,Parazynski Settlement,Ferguson Platform,Kubasov's Inheritance"
+ },
+ {
+ "name": "Ghekreep",
+ "pos_x": -61.84375,
+ "pos_y": 83.03125,
+ "pos_z": 124.34375,
+ "stations": "Townshend Gateway,Ali Station,Haber Dock,Clifford Exchange"
+ },
+ {
+ "name": "Ghimal",
+ "pos_x": 32.9375,
+ "pos_y": 5.8125,
+ "pos_z": 65.5,
+ "stations": "Schweinfurth Penal colony,Xing Dock,Lonchakov Depot,Waldrop Installation"
+ },
+ {
+ "name": "Ghimbo",
+ "pos_x": 14,
+ "pos_y": -171.84375,
+ "pos_z": -63,
+ "stations": "Geston Prospect,Chongzhi Terminal,Kandrup Colony"
+ },
+ {
+ "name": "Ghimr",
+ "pos_x": 17.28125,
+ "pos_y": -80.53125,
+ "pos_z": -20.5,
+ "stations": "Wells Orbital,Santos Prospect,Griffith Installation,Kingsmill Hub,Joule Installation"
+ },
+ {
+ "name": "Giabaing",
+ "pos_x": 56.90625,
+ "pos_y": -158.03125,
+ "pos_z": -27.6875,
+ "stations": "Stephan Enterprise,Jones City,Chandra Hub,Coye Gateway,Gurevich Stop,Fischer Relay"
+ },
+ {
+ "name": "Giabal",
+ "pos_x": 5.5,
+ "pos_y": -175.625,
+ "pos_z": 26,
+ "stations": "Sunyaev Dock,Serre Terminal,Reinmuth Silo"
+ },
+ {
+ "name": "Gienah",
+ "pos_x": 101.9375,
+ "pos_y": 107.375,
+ "pos_z": 40.96875,
+ "stations": "Nourse Orbital"
+ },
+ {
+ "name": "Gigal",
+ "pos_x": 7.75,
+ "pos_y": -157.03125,
+ "pos_z": 99.03125,
+ "stations": "Sitterly Holdings,Sheepshanks Settlement,Karman Survey"
+ },
+ {
+ "name": "Gigaliep",
+ "pos_x": -81.71875,
+ "pos_y": 27.5625,
+ "pos_z": 16.4375,
+ "stations": "Debye Dock,Weston Dock,Petaja Horizons,Margulis Enterprise"
+ },
+ {
+ "name": "Giguara",
+ "pos_x": 13.3125,
+ "pos_y": 14.5,
+ "pos_z": 68.21875,
+ "stations": "Samos Port,Elvstrom Gateway,Atiyah Dock,Tevis City,Neff Port"
+ },
+ {
+ "name": "Giguen",
+ "pos_x": 124.8125,
+ "pos_y": 45.125,
+ "pos_z": 3.71875,
+ "stations": "Helms Orbital,Joule Port,Henry Dock,Scheutz Enterprise,Leibniz Terminal,Brunel Horizons,Ford Depot"
+ },
+ {
+ "name": "Gilgamesh",
+ "pos_x": -59.875,
+ "pos_y": -16,
+ "pos_z": 28.78125,
+ "stations": "Back Works,Moffitt City,Macan Orbital,Daniel Terminal,Bunch City,Scalzi Dock,Clarke Terminal,Mallett Hub,Carpenter's Progress,Lopez de Haro Ring,Penn's Folly,Leichhardt Dock,Liouville Survey,Perez Terminal"
+ },
+ {
+ "name": "Giltine",
+ "pos_x": 39.28125,
+ "pos_y": -125.46875,
+ "pos_z": 22.21875,
+ "stations": "Tanaka Bastion,Wolszczan Orbital,South Orbital"
+ },
+ {
+ "name": "Gilya",
+ "pos_x": -76.78125,
+ "pos_y": 51.03125,
+ "pos_z": 21.75,
+ "stations": "Bell Orbital,Kendrick Enterprise,la Cosa Penal colony"
+ },
+ {
+ "name": "Gilyadar",
+ "pos_x": 39.9375,
+ "pos_y": -101.9375,
+ "pos_z": 61.78125,
+ "stations": "Miller Station"
+ },
+ {
+ "name": "Gilyan",
+ "pos_x": -166.78125,
+ "pos_y": 72.96875,
+ "pos_z": -54.75,
+ "stations": "Laing Sanctuary,Swift Platform,Resnick Barracks"
+ },
+ {
+ "name": "Ginnae",
+ "pos_x": 95.53125,
+ "pos_y": -76.53125,
+ "pos_z": 123.75,
+ "stations": "Bruck Vista,Seamans Port"
+ },
+ {
+ "name": "Ginnari",
+ "pos_x": 103.375,
+ "pos_y": 81,
+ "pos_z": 39.84375,
+ "stations": "Knight Holdings,Wang Depot"
+ },
+ {
+ "name": "Gio",
+ "pos_x": -46.09375,
+ "pos_y": 48.8125,
+ "pos_z": 119.09375,
+ "stations": "Lockhart City,Drew Mines,Caselberg Settlement,Issigonis Enterprise"
+ },
+ {
+ "name": "Gippsworld",
+ "pos_x": 81.46875,
+ "pos_y": 69.65625,
+ "pos_z": 36.03125,
+ "stations": "Jim Bergerac"
+ },
+ {
+ "name": "Gira",
+ "pos_x": 86.96875,
+ "pos_y": -225.09375,
+ "pos_z": 46.5625,
+ "stations": "Keeler Ring"
+ },
+ {
+ "name": "Girantxe",
+ "pos_x": 145.71875,
+ "pos_y": -190.09375,
+ "pos_z": -30.5,
+ "stations": "Krigstein Mine,Foster Escape"
+ },
+ {
+ "name": "Giras",
+ "pos_x": 92.78125,
+ "pos_y": -262.40625,
+ "pos_z": 3.84375,
+ "stations": "Helffrich's Pride,Gould Dock,Blaylock Survey"
+ },
+ {
+ "name": "Girawa",
+ "pos_x": -76.3125,
+ "pos_y": -69.84375,
+ "pos_z": 57.21875,
+ "stations": "Hardy Refinery"
+ },
+ {
+ "name": "Girya Gu",
+ "pos_x": -13.625,
+ "pos_y": -10.625,
+ "pos_z": -75.25,
+ "stations": "Pierce City,George Keep,Gregory Camp"
+ },
+ {
+ "name": "Giryak",
+ "pos_x": 14.6875,
+ "pos_y": 27.65625,
+ "pos_z": 108.65625,
+ "stations": "Pater's Memorial,Watson Orbital,Dunbar Ring,Boas Escape,Ackerman Stop,Polyakov Vista"
+ },
+ {
+ "name": "Giryamba",
+ "pos_x": 104.46875,
+ "pos_y": 41.96875,
+ "pos_z": -157.84375,
+ "stations": "Lefschetz Orbital"
+ },
+ {
+ "name": "Giryampi",
+ "pos_x": 121.96875,
+ "pos_y": -78.96875,
+ "pos_z": 11.03125,
+ "stations": "Cottenot Terminal,Whipple Terminal,Chaffee Terminal"
+ },
+ {
+ "name": "Gitsaugusi",
+ "pos_x": -53.125,
+ "pos_y": 34.53125,
+ "pos_z": 157.625,
+ "stations": "Strekalov Enterprise,Szentmartony Vision,Laliberte Landing"
+ },
+ {
+ "name": "Gitse",
+ "pos_x": 50.40625,
+ "pos_y": 5.90625,
+ "pos_z": -53.0625,
+ "stations": "White Ring,Slayton Market,Lichtenberg Hub"
+ },
+ {
+ "name": "Gitxsan",
+ "pos_x": 68.5,
+ "pos_y": -72.53125,
+ "pos_z": 26.40625,
+ "stations": "Stephan Orbital"
+ },
+ {
+ "name": "Gitxsangni",
+ "pos_x": 111.5,
+ "pos_y": -36.03125,
+ "pos_z": 69.96875,
+ "stations": "Petaja Dock"
+ },
+ {
+ "name": "Gitxsanluga",
+ "pos_x": 26,
+ "pos_y": -93.1875,
+ "pos_z": -102.34375,
+ "stations": "Weitz Enterprise,Kopra Station,Gardner City,Carver Enterprise,Chiao Relay,Petaja Holdings,Ozanne Terminal"
+ },
+ {
+ "name": "Gitxsante",
+ "pos_x": 6.125,
+ "pos_y": -10.78125,
+ "pos_z": -138.875,
+ "stations": "Szentmartony Dock,Rowley Terminal,Peral Survey,Morgan Colony"
+ },
+ {
+ "name": "Gjalis",
+ "pos_x": 126.3125,
+ "pos_y": -148.09375,
+ "pos_z": -38.15625,
+ "stations": "Pennington Port,Mozhaysky Outpost,Harrison Relay"
+ },
+ {
+ "name": "Gkutat",
+ "pos_x": 105.25,
+ "pos_y": -13.8125,
+ "pos_z": -72.4375,
+ "stations": "Beltrami Prospect,Martin Hangar"
+ },
+ {
+ "name": "Gkuthangli",
+ "pos_x": 111.0625,
+ "pos_y": 43.0625,
+ "pos_z": 0.46875,
+ "stations": "Resnik Mines,Springer Beacon"
+ },
+ {
+ "name": "Gl 550.1",
+ "pos_x": -24.1875,
+ "pos_y": 121,
+ "pos_z": 42.34375,
+ "stations": "Ostrander's Pride"
+ },
+ {
+ "name": "Gl 606.1 B",
+ "pos_x": 60.71875,
+ "pos_y": -33.125,
+ "pos_z": 46.96875,
+ "stations": "Harvey Ring,Ohm Horizons,Stefanyshyn-Piper Terminal"
+ },
+ {
+ "name": "Gl 776.1",
+ "pos_x": 12.6875,
+ "pos_y": -73.8125,
+ "pos_z": 120.40625,
+ "stations": "Goldschmidt Installation,Peary Installation,Lozino-Lozinskiy Prospect,Rutan Terminal"
+ },
+ {
+ "name": "Gladgudiya",
+ "pos_x": 143.375,
+ "pos_y": -84.9375,
+ "pos_z": 116.875,
+ "stations": "Fu Lab,Ayers Dock"
+ },
+ {
+ "name": "Gladutjaras",
+ "pos_x": 106.84375,
+ "pos_y": -195.59375,
+ "pos_z": 99.875,
+ "stations": "Faber Platform,Tuttle Horizons,Samos Barracks"
+ },
+ {
+ "name": "Gladutjin",
+ "pos_x": -40.90625,
+ "pos_y": 11.84375,
+ "pos_z": -55.8125,
+ "stations": "Chadwick Port,Lounge Hub,Serebrov Dock"
+ },
+ {
+ "name": "Gladuwong",
+ "pos_x": 40.25,
+ "pos_y": -180.6875,
+ "pos_z": -30.40625,
+ "stations": "Lubbock Terminal"
+ },
+ {
+ "name": "Gladyak",
+ "pos_x": -33.75,
+ "pos_y": 94.71875,
+ "pos_z": 31.78125,
+ "stations": "Anthony Terminal,Holdstock Orbital,Houtman Colony,Collins Plant"
+ },
+ {
+ "name": "Gladyangar",
+ "pos_x": 2.1875,
+ "pos_y": -47.09375,
+ "pos_z": 131.625,
+ "stations": "Debye Dock,Boodt Base,Hurley Landing,Guerrero Keep"
+ },
+ {
+ "name": "Glaucus",
+ "pos_x": -65.96875,
+ "pos_y": -32.9375,
+ "pos_z": -33.28125,
+ "stations": "Knapp Terminal,Mourelle Relay"
+ },
+ {
+ "name": "Glauss",
+ "pos_x": -15.15625,
+ "pos_y": -16,
+ "pos_z": 98.625,
+ "stations": "Cori Terminal,Karl Diesel Dock,Chandler Keep,Oswald Market,Banks Horizons,Hutchinson Base"
+ },
+ {
+ "name": "Glete",
+ "pos_x": -30.125,
+ "pos_y": -99.71875,
+ "pos_z": -98.71875,
+ "stations": "May's Pride,Betancourt Base"
+ },
+ {
+ "name": "Gletichua",
+ "pos_x": -66.4375,
+ "pos_y": -59.03125,
+ "pos_z": -125.125,
+ "stations": "Nasmyth Base,Usachov Terminal"
+ },
+ {
+ "name": "Gletine",
+ "pos_x": 34.3125,
+ "pos_y": -133.59375,
+ "pos_z": 98.34375,
+ "stations": "Mohr Penal colony,Flammarion Station,Alphonsi Colony"
+ },
+ {
+ "name": "Gliese 105.2",
+ "pos_x": 85.40625,
+ "pos_y": -178.5625,
+ "pos_z": -25.78125,
+ "stations": "Payne-Gaposchkin Horizons,Donati Station,Fokker Dock,Ray Arena,Bolton Penal colony"
+ },
+ {
+ "name": "Gliese 110",
+ "pos_x": 115.75,
+ "pos_y": -129.5625,
+ "pos_z": 35.15625,
+ "stations": "Mason Dock,Faber Station,Townes' Progress,Finlay Colony"
+ },
+ {
+ "name": "Gliese 113.1",
+ "pos_x": -59.875,
+ "pos_y": -57.46875,
+ "pos_z": -108.4375,
+ "stations": "Elvstrom Depot,Harrison Colony,Westerfeld Mines"
+ },
+ {
+ "name": "Gliese 1152",
+ "pos_x": -2.1875,
+ "pos_y": 157.9375,
+ "pos_z": -40.8125,
+ "stations": "Teller Colony,Clifford Relay"
+ },
+ {
+ "name": "Gliese 1272",
+ "pos_x": -100.96875,
+ "pos_y": -89.34375,
+ "pos_z": 15.9375,
+ "stations": "Gregory Enterprise,Bao Dock,Mitropoulos Prospect,Dickson Port,Murphy Dock,Barnes Port,Doyle Works,Alexandria Works,Gregory Terminal,Maler Station,Schmidt Port,Gerlache Horizons,Sylvester Barracks"
+ },
+ {
+ "name": "Gliese 138.1",
+ "pos_x": -14.71875,
+ "pos_y": -104.40625,
+ "pos_z": -131.4375,
+ "stations": "Thomas Mine,Vetulani Base"
+ },
+ {
+ "name": "Gliese 170.1",
+ "pos_x": 1.71875,
+ "pos_y": -50.15625,
+ "pos_z": -131.65625,
+ "stations": "Foden Dock,Arber Penal colony"
+ },
+ {
+ "name": "Gliese 182.1",
+ "pos_x": 14.0625,
+ "pos_y": -34.21875,
+ "pos_z": -117.5,
+ "stations": "Schmidt Hub,Klink Escape,Knight Installation"
+ },
+ {
+ "name": "Gliese 2067",
+ "pos_x": 85.4375,
+ "pos_y": 43.375,
+ "pos_z": -86.09375,
+ "stations": "Brunton Station,Burroughs Survey"
+ },
+ {
+ "name": "Gliese 2084",
+ "pos_x": 120.1875,
+ "pos_y": 81.40625,
+ "pos_z": 19.34375,
+ "stations": "Feynman Dock,Hudson Depot,Barratt Landing"
+ },
+ {
+ "name": "Gliese 2119",
+ "pos_x": 54.46875,
+ "pos_y": 5.5625,
+ "pos_z": 116.5625,
+ "stations": "Humphreys Hub,Shepard Vision,Sellings' Progress,Slade Settlement,Hawkes Vision"
+ },
+ {
+ "name": "Gliese 2126",
+ "pos_x": 20.125,
+ "pos_y": 4.75,
+ "pos_z": 123.375,
+ "stations": "Tanner Arena,Benyovszky Escape"
+ },
+ {
+ "name": "Gliese 225.1",
+ "pos_x": 134.15625,
+ "pos_y": -71.0625,
+ "pos_z": -67.28125,
+ "stations": "Zander Prospect,Hoyle Terminal,Sunyaev Orbital,Andree Forum"
+ },
+ {
+ "name": "Gliese 3002",
+ "pos_x": -104.90625,
+ "pos_y": -58.1875,
+ "pos_z": -44.15625,
+ "stations": "Vizcaino Terminal,Bushkov Installation,Coelho Ring,Hamilton Port,Rucker Gateway"
+ },
+ {
+ "name": "Gliese 307",
+ "pos_x": 48.34375,
+ "pos_y": 75.34375,
+ "pos_z": -116.25,
+ "stations": "Dirac Settlement,Gemar Relay"
+ },
+ {
+ "name": "Gliese 3095",
+ "pos_x": -58.25,
+ "pos_y": -119.65625,
+ "pos_z": -63.5625,
+ "stations": "Rennie Station,Grunsfeld City,Scheutz Station"
+ },
+ {
+ "name": "Gliese 3206",
+ "pos_x": -86.375,
+ "pos_y": 8.03125,
+ "pos_z": -100.09375,
+ "stations": "Vercors Vision,Pike Colony"
+ },
+ {
+ "name": "Gliese 3244",
+ "pos_x": 18.59375,
+ "pos_y": -104.6875,
+ "pos_z": -115.09375,
+ "stations": "Oltion Base,Stasheff Base"
+ },
+ {
+ "name": "Gliese 3251",
+ "pos_x": -75.125,
+ "pos_y": 16.25,
+ "pos_z": -95.21875,
+ "stations": "Lister Colony,Ohm Survey"
+ },
+ {
+ "name": "Gliese 3258",
+ "pos_x": 57.90625,
+ "pos_y": -98.59375,
+ "pos_z": -66.59375,
+ "stations": "Syromyatnikov Vision,Hind Ring,Wild Terminal,Tsiolkovsky Ring,Lemaitre Station,Wirtanen Gateway,Michael's Folly,Stiegler Holdings,Kempf Beacon"
+ },
+ {
+ "name": "Gliese 3299",
+ "pos_x": -34.46875,
+ "pos_y": -10.9375,
+ "pos_z": -116.125,
+ "stations": "Brandenstein Terminal,Haber Ring"
+ },
+ {
+ "name": "Gliese 3318",
+ "pos_x": 71.65625,
+ "pos_y": -75.34375,
+ "pos_z": -84.75,
+ "stations": "Andersson Keep,Wafer Colony"
+ },
+ {
+ "name": "Gliese 3371",
+ "pos_x": -17.71875,
+ "pos_y": 12.96875,
+ "pos_z": -38.3125,
+ "stations": "Barry Gateway,Precourt Port"
+ },
+ {
+ "name": "Gliese 3680",
+ "pos_x": 116.25,
+ "pos_y": 72.90625,
+ "pos_z": 32.65625,
+ "stations": "Parmitano Horizons"
+ },
+ {
+ "name": "Gliese 3761",
+ "pos_x": 111.90625,
+ "pos_y": -35.59375,
+ "pos_z": 75.71875,
+ "stations": "Meech Horizons,Lewis Port"
+ },
+ {
+ "name": "Gliese 384",
+ "pos_x": 127.53125,
+ "pos_y": 16.46875,
+ "pos_z": 17,
+ "stations": "He Station,Kennedy Silo,Naddoddur's Progress,Zettel Station,Scott Station,Fourier Prospect,Cremona Terminal,Zoline Enterprise,Martinez Enterprise,Phillips Vision"
+ },
+ {
+ "name": "Gliese 3854",
+ "pos_x": 42.75,
+ "pos_y": 89.40625,
+ "pos_z": 96.28125,
+ "stations": "Melvill Vision,Kregel Survey"
+ },
+ {
+ "name": "Gliese 3857",
+ "pos_x": 4.5625,
+ "pos_y": 112.96875,
+ "pos_z": 72.5,
+ "stations": "Cochrane Observatory,Boyle Hub,Almagro Survey"
+ },
+ {
+ "name": "Gliese 3876",
+ "pos_x": -103.1875,
+ "pos_y": 78.5,
+ "pos_z": -55.9375,
+ "stations": "Zalyotin Mines,Mieville Vision"
+ },
+ {
+ "name": "Gliese 3897 B",
+ "pos_x": -38.375,
+ "pos_y": 126.03125,
+ "pos_z": 75.3125,
+ "stations": "Seega Ring"
+ },
+ {
+ "name": "Gliese 3944",
+ "pos_x": 12.65625,
+ "pos_y": 43.53125,
+ "pos_z": 115.65625,
+ "stations": "Watson Landing,Shalatula Lab,Dyson Terminal"
+ },
+ {
+ "name": "Gliese 4014",
+ "pos_x": 41.125,
+ "pos_y": -22.96875,
+ "pos_z": 185.5,
+ "stations": "Kimberlin Reach"
+ },
+ {
+ "name": "Gliese 4052",
+ "pos_x": -44.0625,
+ "pos_y": 4.0625,
+ "pos_z": 113.75,
+ "stations": "Trevithick Orbital,Reed Arsenal"
+ },
+ {
+ "name": "Gliese 4261",
+ "pos_x": -165.65625,
+ "pos_y": -94.53125,
+ "pos_z": 30.9375,
+ "stations": "Bass Landing,Schouten Hub"
+ },
+ {
+ "name": "Gliese 4328",
+ "pos_x": -82.84375,
+ "pos_y": -88.1875,
+ "pos_z": 1.46875,
+ "stations": "Chaudhary Base"
+ },
+ {
+ "name": "Gliese 4366",
+ "pos_x": -80.15625,
+ "pos_y": -121.96875,
+ "pos_z": -7.8125,
+ "stations": "Rozhdestvensky Orbital,Ray Laboratory,Burbank Survey"
+ },
+ {
+ "name": "Gliese 44",
+ "pos_x": -50.375,
+ "pos_y": -132.6875,
+ "pos_z": -39.09375,
+ "stations": "Rizvi Orbital,Wilhelm Silo,Lorrah Camp"
+ },
+ {
+ "name": "Gliese 452.3",
+ "pos_x": 30,
+ "pos_y": 127.6875,
+ "pos_z": -15.5,
+ "stations": "Bowen Vision,Leeuwenhoek Orbital"
+ },
+ {
+ "name": "Gliese 460",
+ "pos_x": 16.5625,
+ "pos_y": 208.90625,
+ "pos_z": -17.40625,
+ "stations": "Ashton Works"
+ },
+ {
+ "name": "Gliese 488.2",
+ "pos_x": 54.875,
+ "pos_y": 100.75,
+ "pos_z": 37.75,
+ "stations": "Cugnot Horizons,Rotsler Installation,Onufriyenko Platform,Humboldt Dock,Russell Survey"
+ },
+ {
+ "name": "Gliese 506.2",
+ "pos_x": -121.625,
+ "pos_y": 92.03125,
+ "pos_z": -76.84375,
+ "stations": "Dietz Orbital,Adams Enterprise"
+ },
+ {
+ "name": "Gliese 550",
+ "pos_x": 82.34375,
+ "pos_y": 16.8125,
+ "pos_z": 91.5625,
+ "stations": "Wyndham Port,Rawat Port,Cochrane Hub,Akers Vista,Cousteau Enterprise,Doctorow Terminal"
+ },
+ {
+ "name": "Gliese 563.1",
+ "pos_x": -32.5625,
+ "pos_y": 70.21875,
+ "pos_z": 15.40625,
+ "stations": "Matteucci Ring,Brand Enterprise"
+ },
+ {
+ "name": "Gliese 577",
+ "pos_x": -96.875,
+ "pos_y": 107.25,
+ "pos_z": -20.125,
+ "stations": "Quimper Landing,Wyndham Refinery"
+ },
+ {
+ "name": "Gliese 58",
+ "pos_x": 11.125,
+ "pos_y": -128.90625,
+ "pos_z": -14.15625,
+ "stations": "Roth Station,Baillaud Dock,Biermann Vision,Maanen Settlement,Orbik Ring,Maunder's Folly,Delaunay Orbital"
+ },
+ {
+ "name": "Gliese 599.1",
+ "pos_x": 70.53125,
+ "pos_y": 5.34375,
+ "pos_z": 121.6875,
+ "stations": "Arnarson Hub,Lessing City,Morrison Landing,Daniel Station,Bethke Barracks"
+ },
+ {
+ "name": "Gliese 647",
+ "pos_x": -61.875,
+ "pos_y": 67.71875,
+ "pos_z": 96.40625,
+ "stations": "Ivanishin Dock,Midgeley Base"
+ },
+ {
+ "name": "Gliese 668.1",
+ "pos_x": -45.25,
+ "pos_y": 46.46875,
+ "pos_z": 148.46875,
+ "stations": "Rice Beacon,Wallin Port,Shawl Port"
+ },
+ {
+ "name": "Gliese 67.2",
+ "pos_x": 91.34375,
+ "pos_y": -241.5625,
+ "pos_z": 10.125,
+ "stations": "Curtiss Silo,Urata Colony,Palisa Terminal"
+ },
+ {
+ "name": "Gliese 683.2",
+ "pos_x": 21.8125,
+ "pos_y": -10.625,
+ "pos_z": 153.25,
+ "stations": "McQuay Escape,Roberts Landing"
+ },
+ {
+ "name": "Gliese 724.2",
+ "pos_x": 82.90625,
+ "pos_y": -60.65625,
+ "pos_z": 87.65625,
+ "stations": "d'Allonville Port,Kopal Gateway,Barcelona City,Finlay-Freundlich Prospect,Young Survey"
+ },
+ {
+ "name": "Gliese 76",
+ "pos_x": 16.84375,
+ "pos_y": -131.75,
+ "pos_z": -25.5,
+ "stations": "Thorne Hub,Rond d'Alembert Arsenal,Huss Prospect,Bohr Station"
+ },
+ {
+ "name": "Gliese 765.3",
+ "pos_x": -138.4375,
+ "pos_y": 40.375,
+ "pos_z": -1.375,
+ "stations": "Ham City,Brendan Enterprise,Saberhagen Base,Czerny Port,Sarich Camp"
+ },
+ {
+ "name": "Gliese 828.4",
+ "pos_x": -114,
+ "pos_y": -68.28125,
+ "pos_z": 55.6875,
+ "stations": "Wundt Terminal"
+ },
+ {
+ "name": "Gliese 851.3",
+ "pos_x": -51.46875,
+ "pos_y": -98.53125,
+ "pos_z": 55.46875,
+ "stations": "Conrad's Claim,Viehbock Installation,Sherrington Survey"
+ },
+ {
+ "name": "Gliese 867.1",
+ "pos_x": -53.375,
+ "pos_y": -100.9375,
+ "pos_z": 40.84375,
+ "stations": "Friesner Port,Holdstock Station,Saunders Hub,Minkowski Terminal,Sohl Station,Barnes City,Griffin Station,Holberg Hub,King Relay,Nemere Keep,Flinders Dock,Springer Base,Stott Vision,Mohmand Escape,von Bellingshausen Colony,Waldrop Palace"
+ },
+ {
+ "name": "Gliese 868",
+ "pos_x": -7.375,
+ "pos_y": -38.96875,
+ "pos_z": 19.5,
+ "stations": "MacLean Terminal,Massimino Orbital,Houssay Terminal,Bacon Port,Musgrave Enterprise,Wnuk-Lipinski Terminal,Alas Station,Herreshoff Prospect,Braun Station,Hannu Works,Reilly Enterprise,Russell's Inheritance,Wrangell Terminal,Phillips Horizons,Einstein Arsenal"
+ },
+ {
+ "name": "Gliese 875",
+ "pos_x": -23.4375,
+ "pos_y": -37.90625,
+ "pos_z": 11.75,
+ "stations": "Soddy Settlement,Wilson Hangar,Alexandria Silo"
+ },
+ {
+ "name": "Gliese 900.1",
+ "pos_x": 21.625,
+ "pos_y": -111.5625,
+ "pos_z": 45.40625,
+ "stations": "Ford Dock,Barr Station,Lewitt Port,Leckie Gateway,Peebles Lab,Albitzky Stop,Paez Terminal,Fontenay Hub,RenenBellot Port,Frobisher Oasis,Porubcan Vision,Anderson's Progress,Macgregor Dock,Serre Reach,Amundsen Port"
+ },
+ {
+ "name": "Gliese 9016",
+ "pos_x": -122.96875,
+ "pos_y": -37.21875,
+ "pos_z": -72.40625,
+ "stations": "Baudry Vision,Ziemianski's Folly,Ochoa Hub"
+ },
+ {
+ "name": "Gliese 9029",
+ "pos_x": 21.625,
+ "pos_y": -156.84375,
+ "pos_z": 15.03125,
+ "stations": "Fox City,Milne Orbital,Lewis Station,Rizvi Dock,Mooz Orbital,Hansteen Vision,Nilson Gateway,Kranz City,Watson Dock,Paola Station,Krenkel Refinery,Payne Bastion"
+ },
+ {
+ "name": "Gliese 9035",
+ "pos_x": -116.5,
+ "pos_y": 49.5,
+ "pos_z": -77.21875,
+ "stations": "Kozin's Folly,Anderson Survey,Akers' Inheritance"
+ },
+ {
+ "name": "Gliese 9043",
+ "pos_x": 15,
+ "pos_y": -159.6875,
+ "pos_z": -4.5625,
+ "stations": "Anderson Installation,Murakami Terminal"
+ },
+ {
+ "name": "Gliese 9073",
+ "pos_x": -20.78125,
+ "pos_y": -101.375,
+ "pos_z": -61.8125,
+ "stations": "Gresley Orbital,Stebler Gateway,Bunsen Silo"
+ },
+ {
+ "name": "Gliese 9084",
+ "pos_x": 94.5,
+ "pos_y": -180.1875,
+ "pos_z": -10.46875,
+ "stations": "Wilhelm Enterprise,Cassini Vision,Cartier Mines,Draper Settlement"
+ },
+ {
+ "name": "Gliese 9086",
+ "pos_x": 123.15625,
+ "pos_y": -222.75,
+ "pos_z": -12.0625,
+ "stations": "Al-Khujandi Terminal,Barmin Hub,Lewis Landing"
+ },
+ {
+ "name": "Gliese 9090",
+ "pos_x": 52.875,
+ "pos_y": -193.1875,
+ "pos_z": -70.59375,
+ "stations": "Curtis Settlement"
+ },
+ {
+ "name": "Gliese 9106",
+ "pos_x": -32,
+ "pos_y": -90.90625,
+ "pos_z": -109.96875,
+ "stations": "Abernathy City,McKay Holdings,Phillips Port,Berezin Base"
+ },
+ {
+ "name": "Gliese 9119",
+ "pos_x": -47.9375,
+ "pos_y": -41.03125,
+ "pos_z": -126.40625,
+ "stations": "Ansari Station"
+ },
+ {
+ "name": "Gliese 9142",
+ "pos_x": 20.46875,
+ "pos_y": -77.8125,
+ "pos_z": -106.78125,
+ "stations": "Bordage Vision,White Installation,Tevis Holdings,Hudson Lab"
+ },
+ {
+ "name": "Gliese 9143",
+ "pos_x": 71.53125,
+ "pos_y": -106.96875,
+ "pos_z": -70.9375,
+ "stations": "Anderson Port,Anderson Penal colony"
+ },
+ {
+ "name": "Gliese 9146",
+ "pos_x": 104.875,
+ "pos_y": -126.75,
+ "pos_z": -50.03125,
+ "stations": "Tombaugh Mines,Penrose Relay"
+ },
+ {
+ "name": "Gliese 9152",
+ "pos_x": 77.75,
+ "pos_y": -87.96875,
+ "pos_z": -37.09375,
+ "stations": "Ivens Survey"
+ },
+ {
+ "name": "Gliese 9204",
+ "pos_x": 104.03125,
+ "pos_y": -50.6875,
+ "pos_z": -40.28125,
+ "stations": "Harvey Terminal,Franklin Outpost"
+ },
+ {
+ "name": "Gliese 9224",
+ "pos_x": 143.65625,
+ "pos_y": -45.28125,
+ "pos_z": -29.875,
+ "stations": "Cheli Refinery,Melnick Installation,Hughes-Fulford Mine"
+ },
+ {
+ "name": "Gliese 9229",
+ "pos_x": 171.4375,
+ "pos_y": -41.1875,
+ "pos_z": -53.1875,
+ "stations": "Parsons Mines,Feoktistov Mines"
+ },
+ {
+ "name": "Gliese 9239",
+ "pos_x": 53.625,
+ "pos_y": 53.15625,
+ "pos_z": -140.75,
+ "stations": "Bretnor Terminal,Benford Horizons,Russell Arena"
+ },
+ {
+ "name": "Gliese 9268",
+ "pos_x": 128.1875,
+ "pos_y": -13.78125,
+ "pos_z": -5.5,
+ "stations": "Yaping Platform,Locke Colony"
+ },
+ {
+ "name": "Gliese 9312",
+ "pos_x": 51.0625,
+ "pos_y": 106.34375,
+ "pos_z": -68.25,
+ "stations": "Wingqvist Plant"
+ },
+ {
+ "name": "Gliese 9318",
+ "pos_x": 139,
+ "pos_y": 39.15625,
+ "pos_z": 1.9375,
+ "stations": "Precourt Mine"
+ },
+ {
+ "name": "Gliese 9336",
+ "pos_x": 137.46875,
+ "pos_y": 94.0625,
+ "pos_z": -0.8125,
+ "stations": "Belyanin Vision,Delany Oasis"
+ },
+ {
+ "name": "Gliese 9378 B",
+ "pos_x": 28.40625,
+ "pos_y": 121.03125,
+ "pos_z": -14.6875,
+ "stations": "Malyshev Ring,Nicollier Hub,Brash Relay"
+ },
+ {
+ "name": "Gliese 9392",
+ "pos_x": 55.59375,
+ "pos_y": 127.28125,
+ "pos_z": 8.125,
+ "stations": "Ellis Enterprise,Mallory's Folly,Balandin Orbital,Fisher Station"
+ },
+ {
+ "name": "Gliese 9407",
+ "pos_x": 105.78125,
+ "pos_y": 73.65625,
+ "pos_z": 56.34375,
+ "stations": "Buffett Relay"
+ },
+ {
+ "name": "Gliese 9408",
+ "pos_x": 75,
+ "pos_y": 135.84375,
+ "pos_z": 32.84375,
+ "stations": "Lorrah Works,Ehrenfried Kegel Beacon"
+ },
+ {
+ "name": "Gliese 9423",
+ "pos_x": 102.6875,
+ "pos_y": 41.34375,
+ "pos_z": 69.59375,
+ "stations": "Salgari Installation,Erikson Hub,Gunn Enterprise,Bierce's Inheritance,Ogden Enterprise,Low Enterprise"
+ },
+ {
+ "name": "Gliese 9468",
+ "pos_x": 113.40625,
+ "pos_y": -1.15625,
+ "pos_z": 103.9375,
+ "stations": "Daniel Landing"
+ },
+ {
+ "name": "Gliese 9480",
+ "pos_x": -27.96875,
+ "pos_y": 137.125,
+ "pos_z": 49.46875,
+ "stations": "Garcia Installation,Euthymenes Settlement,Fozard Penal colony,Thompson Settlement"
+ },
+ {
+ "name": "Gliese 9532",
+ "pos_x": -108.1875,
+ "pos_y": 101.3125,
+ "pos_z": -13.15625,
+ "stations": "Stevens Terminal,Ziemianski Vision,Mohun Survey,Rothfuss Terminal,Navigator Orbital"
+ },
+ {
+ "name": "Gliese 9539",
+ "pos_x": 19.28125,
+ "pos_y": 53.34375,
+ "pos_z": 132.125,
+ "stations": "Gold Hub,Evans Bastion"
+ },
+ {
+ "name": "Gliese 9547",
+ "pos_x": 69.4375,
+ "pos_y": -4.03125,
+ "pos_z": 124.40625,
+ "stations": "Kraepelin City,Jacquard Hub,Cleve Hub"
+ },
+ {
+ "name": "Gliese 9553",
+ "pos_x": 23.3125,
+ "pos_y": 33.5,
+ "pos_z": 123.6875,
+ "stations": "O'Connor City,Humphreys Barracks"
+ },
+ {
+ "name": "Gliese 9735",
+ "pos_x": 31.1875,
+ "pos_y": -136.96875,
+ "pos_z": 135.6875,
+ "stations": "Lindblad Colony,Juan de la Cierva Prospect"
+ },
+ {
+ "name": "Gline",
+ "pos_x": 24.25,
+ "pos_y": -76.5,
+ "pos_z": -101.65625,
+ "stations": "Sleator Relay,Melnick Vision,Crick Works"
+ },
+ {
+ "name": "Glinja",
+ "pos_x": -124.1875,
+ "pos_y": 18.34375,
+ "pos_z": 77.53125,
+ "stations": "Bugrov Outpost,Russ Survey"
+ },
+ {
+ "name": "Glooscap",
+ "pos_x": -126.6875,
+ "pos_y": 10.53125,
+ "pos_z": 3.625,
+ "stations": "Culpeper Gateway,Shaw Hub,Forstchen Terminal"
+ },
+ {
+ "name": "Glusargiz",
+ "pos_x": -39.28125,
+ "pos_y": -114.21875,
+ "pos_z": 21.125,
+ "stations": "Oren Dock,Fraknoi Terminal,Bauman Dock,Hevelius Terminal,Alfven Terminal,Kirby Hub,Chacornac Hub,Sutter Vision,Tall Point"
+ },
+ {
+ "name": "Gluscab",
+ "pos_x": 17.65625,
+ "pos_y": -123.9375,
+ "pos_z": 160.375,
+ "stations": "Murakami Dock,Niijima Station,van Houten Terminal,Kneale Installation,Roe Depot"
+ },
+ {
+ "name": "Gluscabi",
+ "pos_x": 20.65625,
+ "pos_y": -235.78125,
+ "pos_z": -15.5625,
+ "stations": "Robert Platform"
+ },
+ {
+ "name": "Gluscap",
+ "pos_x": 80.78125,
+ "pos_y": 15.28125,
+ "pos_z": 28.09375,
+ "stations": "Clark Hub,Morey Orbital,Vian Station"
+ },
+ {
+ "name": "Glusche",
+ "pos_x": 141.875,
+ "pos_y": 57.78125,
+ "pos_z": -63.6875,
+ "stations": "Hart Vision,Irens Vista"
+ },
+ {
+ "name": "Gluscheimr",
+ "pos_x": 53.9375,
+ "pos_y": -5.78125,
+ "pos_z": -36.625,
+ "stations": "Jemison Orbital,Prunariu Terminal"
+ },
+ {
+ "name": "Gluschen",
+ "pos_x": 131.875,
+ "pos_y": -173.90625,
+ "pos_z": -100,
+ "stations": "Siddha Vision,Vaucouleurs Camp"
+ },
+ {
+ "name": "Gluskabiku",
+ "pos_x": -129.3125,
+ "pos_y": 13.78125,
+ "pos_z": -61.03125,
+ "stations": "Peary Port"
+ },
+ {
+ "name": "Gluskaiabal",
+ "pos_x": -85.40625,
+ "pos_y": -132.96875,
+ "pos_z": -61,
+ "stations": "Cartan Orbital,Piccard Platform,Rotsler Orbital,Rodrigues Base,Diophantus Enterprise,Maybury's Folly"
+ },
+ {
+ "name": "Gluskapuru",
+ "pos_x": 15.78125,
+ "pos_y": -5.40625,
+ "pos_z": -135.875,
+ "stations": "Brothers Point"
+ },
+ {
+ "name": "Gluskas",
+ "pos_x": -58.3125,
+ "pos_y": 34.53125,
+ "pos_z": 161.25,
+ "stations": "Carlisle Dock,Donaldson Ring,Whymper Colony,Barjavel Vision,O'Brien Base"
+ },
+ {
+ "name": "Glusu",
+ "pos_x": -84.96875,
+ "pos_y": 43.28125,
+ "pos_z": 124.25,
+ "stations": "Locke Hub,Birdseye Station,Carpenter Orbital,Vancouver Penal colony"
+ },
+ {
+ "name": "Glutia",
+ "pos_x": 51.09375,
+ "pos_y": -75.34375,
+ "pos_z": -24.375,
+ "stations": "Powers Terminal,Windt Hangar"
+ },
+ {
+ "name": "GM Cephei",
+ "pos_x": -2660.96875,
+ "pos_y": 180.15625,
+ "pos_z": -433.15625,
+ "stations": "Elephant's Trunk Mine"
+ },
+ {
+ "name": "Gnowee",
+ "pos_x": 41.46875,
+ "pos_y": 9.03125,
+ "pos_z": 29.5625,
+ "stations": "Kelleam Port,Abasheli City"
+ },
+ {
+ "name": "Goanna",
+ "pos_x": 81.125,
+ "pos_y": 46.25,
+ "pos_z": -132.25,
+ "stations": "Ross Point"
+ },
+ {
+ "name": "Goansi",
+ "pos_x": 123.53125,
+ "pos_y": 3.84375,
+ "pos_z": -104.96875,
+ "stations": "Bokeili Port,Grassmann Port,Dupuy de Lome Horizons,Fincke Bastion"
+ },
+ {
+ "name": "Godharing",
+ "pos_x": 110.59375,
+ "pos_y": 49.78125,
+ "pos_z": -31.46875,
+ "stations": "Fast Freeport,Kettle Dock"
+ },
+ {
+ "name": "Godhay",
+ "pos_x": 82.34375,
+ "pos_y": 82.375,
+ "pos_z": 110.0625,
+ "stations": "Behring Station"
+ },
+ {
+ "name": "Goeng Gong",
+ "pos_x": 153.59375,
+ "pos_y": -109.78125,
+ "pos_z": 32.625,
+ "stations": "Ryan Vision,Denning Terminal,Rees Station,Abetti Station,Gold Vision,Tusi Dock"
+ },
+ {
+ "name": "Goenpeiadi",
+ "pos_x": -24,
+ "pos_y": -132.15625,
+ "pos_z": 82.4375,
+ "stations": "van de Hulst Station,Giclas Settlement,Schwarzschild Landing"
+ },
+ {
+ "name": "Gogonir",
+ "pos_x": -133.65625,
+ "pos_y": -22.03125,
+ "pos_z": 74.90625,
+ "stations": "Friesner Colony"
+ },
+ {
+ "name": "Goibniu",
+ "pos_x": -54.75,
+ "pos_y": 150.59375,
+ "pos_z": 81.09375,
+ "stations": "Farmer Colony"
+ },
+ {
+ "name": "Goibniugo",
+ "pos_x": 140.9375,
+ "pos_y": 1.71875,
+ "pos_z": 45.8125,
+ "stations": "Magnus Hub,Noon Orbital"
+ },
+ {
+ "name": "Goll",
+ "pos_x": -2.1875,
+ "pos_y": -26.1875,
+ "pos_z": 134.78125,
+ "stations": "Kwolek Platform,MacVicar Prospect,Oersted Camp"
+ },
+ {
+ "name": "Golle",
+ "pos_x": 103.65625,
+ "pos_y": -162.46875,
+ "pos_z": 135.65625,
+ "stations": "Heinemann Camp"
+ },
+ {
+ "name": "Goller",
+ "pos_x": 125.21875,
+ "pos_y": -65.0625,
+ "pos_z": 2.875,
+ "stations": "Ban Enterprise,Grushin Laboratory,Shaw Port"
+ },
+ {
+ "name": "Gollobo",
+ "pos_x": 6.6875,
+ "pos_y": 0.5625,
+ "pos_z": -123.34375,
+ "stations": "Blair Dock"
+ },
+ {
+ "name": "Gomam",
+ "pos_x": -136.0625,
+ "pos_y": -96.71875,
+ "pos_z": -19.375,
+ "stations": "Sternberg Landing,Brunner Installation,Sheckley Landing"
+ },
+ {
+ "name": "Goman",
+ "pos_x": 151.875,
+ "pos_y": -173.78125,
+ "pos_z": 25.28125,
+ "stations": "Gustav Sporer Port"
+ },
+ {
+ "name": "Gomeisa",
+ "pos_x": 78.8125,
+ "pos_y": 34.25,
+ "pos_z": -137,
+ "stations": "Fidalgo Penal colony,Barcelos Lab"
+ },
+ {
+ "name": "Gomm Cail",
+ "pos_x": 21.09375,
+ "pos_y": 24.40625,
+ "pos_z": 88.15625,
+ "stations": "Popovich Terminal,Onufrienko Settlement"
+ },
+ {
+ "name": "Gomm Crua",
+ "pos_x": -41.46875,
+ "pos_y": 27.21875,
+ "pos_z": -51.15625,
+ "stations": "Brunel Hub,Clervoy Enterprise,Dorsett Enterprise,Sinclair Station,Jiushao Arsenal,Mendeleev Station,Foale Base"
+ },
+ {
+ "name": "Gommara",
+ "pos_x": -86.5625,
+ "pos_y": 29.96875,
+ "pos_z": -4.0625,
+ "stations": "Shukor Platform,Virchow Dock"
+ },
+ {
+ "name": "Gommat",
+ "pos_x": 2.9375,
+ "pos_y": 8.59375,
+ "pos_z": -19.53125,
+ "stations": "Cayley Dock"
+ },
+ {
+ "name": "Gommatchal",
+ "pos_x": 150.625,
+ "pos_y": 0.75,
+ "pos_z": 44.21875,
+ "stations": "Peano Point,Heinlein's Folly"
+ },
+ {
+ "name": "Gondan",
+ "pos_x": 7.5625,
+ "pos_y": -193.21875,
+ "pos_z": 5.40625,
+ "stations": "Draper Terminal,Bouwens Dock,Pilcher Mines,Cousteau Installation,Gaughan Gateway,Koishikawa's Progress"
+ },
+ {
+ "name": "Gondul",
+ "pos_x": 66.0625,
+ "pos_y": -109.625,
+ "pos_z": 45.96875,
+ "stations": "Bessel Landing,Deslandres Relay,Fiorilla Dock,Marques Beacon"
+ },
+ {
+ "name": "Gong Biama",
+ "pos_x": 149.8125,
+ "pos_y": -155.3125,
+ "pos_z": 34.96875,
+ "stations": "Vyssotsky Works"
+ },
+ {
+ "name": "Gong Gu",
+ "pos_x": -88.90625,
+ "pos_y": 26.1875,
+ "pos_z": -4.6875,
+ "stations": "Kelly Dock,Klein Vision,Murphy Hub"
+ },
+ {
+ "name": "Gongali",
+ "pos_x": -97.8125,
+ "pos_y": -86.6875,
+ "pos_z": 100.90625,
+ "stations": "Curie Depot"
+ },
+ {
+ "name": "Gongalungul",
+ "pos_x": 76.4375,
+ "pos_y": -85.4375,
+ "pos_z": 27.3125,
+ "stations": "Andoyer Station,Edmondson Port,van der Riet Woolley Ring,Williams' Progress"
+ },
+ {
+ "name": "Gongang",
+ "pos_x": 56.71875,
+ "pos_y": 18.46875,
+ "pos_z": 141.09375,
+ "stations": "Durrance Mines"
+ },
+ {
+ "name": "Gongkates",
+ "pos_x": -44.3125,
+ "pos_y": 39.03125,
+ "pos_z": 142.9375,
+ "stations": "Ivanov Camp"
+ },
+ {
+ "name": "Gooheim",
+ "pos_x": 34.9375,
+ "pos_y": -80.34375,
+ "pos_z": 109.5625,
+ "stations": "Walter Port"
+ },
+ {
+ "name": "Gooheimar",
+ "pos_x": -16.21875,
+ "pos_y": -36.3125,
+ "pos_z": -17.09375,
+ "stations": "Brady Horizons"
+ },
+ {
+ "name": "Goplang",
+ "pos_x": 31.375,
+ "pos_y": -146.34375,
+ "pos_z": 126.125,
+ "stations": "Lindemann Vision,Mayer Orbital,Shirazi Vision,Common Prospect,Benford Hub"
+ },
+ {
+ "name": "Goplatrugba",
+ "pos_x": -154.4375,
+ "pos_y": 49.1875,
+ "pos_z": -46.25,
+ "stations": "Dirac Horizons"
+ },
+ {
+ "name": "Goravas",
+ "pos_x": 58.625,
+ "pos_y": -61.4375,
+ "pos_z": 126.125,
+ "stations": "Lebesgue Enterprise,Lalande Station,von Zach Orbital"
+ },
+ {
+ "name": "Gorengathi",
+ "pos_x": -15.65625,
+ "pos_y": -75.5625,
+ "pos_z": -124.5,
+ "stations": "Pelt Orbital,Fremont Hub"
+ },
+ {
+ "name": "Goreo",
+ "pos_x": -84.34375,
+ "pos_y": 40.71875,
+ "pos_z": 121.1875,
+ "stations": "Quick Outpost,Alvarez de Pineda Refinery"
+ },
+ {
+ "name": "Gores",
+ "pos_x": 134.1875,
+ "pos_y": 98.84375,
+ "pos_z": -34.40625,
+ "stations": "Bear Reformatory"
+ },
+ {
+ "name": "Gorgon",
+ "pos_x": -63.09375,
+ "pos_y": 29.3125,
+ "pos_z": 41.40625,
+ "stations": "Arnason Hub,Ray Hub,Brandenstein Station,Burnet City"
+ },
+ {
+ "name": "Gorimi",
+ "pos_x": -58.125,
+ "pos_y": 10.65625,
+ "pos_z": 129.0625,
+ "stations": "Dana Vision,Melvill Settlement"
+ },
+ {
+ "name": "Gorramacor",
+ "pos_x": 106.90625,
+ "pos_y": -93.125,
+ "pos_z": 49.25,
+ "stations": "Bahcall Settlement"
+ },
+ {
+ "name": "Gorringa",
+ "pos_x": -58.40625,
+ "pos_y": -75.34375,
+ "pos_z": -38.6875,
+ "stations": "Ehrlich Gateway,Readdy's Progress,Bohr Orbital,Alcala Dock"
+ },
+ {
+ "name": "Gorynich",
+ "pos_x": -61.5,
+ "pos_y": -90.46875,
+ "pos_z": 132.25,
+ "stations": "Sturgeon Port"
+ },
+ {
+ "name": "Gorynicnada",
+ "pos_x": 110.8125,
+ "pos_y": -175,
+ "pos_z": 45.90625,
+ "stations": "Couper Depot,Piccard Survey"
+ },
+ {
+ "name": "Gou Jingati",
+ "pos_x": 154.03125,
+ "pos_y": -125.71875,
+ "pos_z": 88.46875,
+ "stations": "Tully Works,Young Barracks"
+ },
+ {
+ "name": "Goudumo",
+ "pos_x": 90.375,
+ "pos_y": -94.09375,
+ "pos_z": -73.375,
+ "stations": "Watanabe Port,McKee Plant,Hickam Vision,Nijland City,Harrison Colony"
+ },
+ {
+ "name": "Gowdalu",
+ "pos_x": 81.3125,
+ "pos_y": 40.3125,
+ "pos_z": -135.8125,
+ "stations": "Kerwin Port,Farrer Gateway,Julian Terminal,Ansari Hub"
+ },
+ {
+ "name": "GQ Virginis",
+ "pos_x": 12.15625,
+ "pos_y": 27.3125,
+ "pos_z": 23.28125,
+ "stations": "Ray Enterprise,Boltzmann Lab,Curie Arsenal"
+ },
+ {
+ "name": "GR 316",
+ "pos_x": 5.34375,
+ "pos_y": -70.84375,
+ "pos_z": -14.4375,
+ "stations": "Sucharitkul Settlement,Hoyle Point"
+ },
+ {
+ "name": "GR Virginis",
+ "pos_x": 26.53125,
+ "pos_y": 119.40625,
+ "pos_z": 113.15625,
+ "stations": "Potagos Ring,Krylov City,Almagro Gateway,Gibbs Bastion"
+ },
+ {
+ "name": "Graba",
+ "pos_x": 68.59375,
+ "pos_y": -41.84375,
+ "pos_z": -62.9375,
+ "stations": "Crick Escape,Bowersox Works"
+ },
+ {
+ "name": "Grabi",
+ "pos_x": 60.9375,
+ "pos_y": -75.25,
+ "pos_z": 10.875,
+ "stations": "Jones Mine"
+ },
+ {
+ "name": "Grabika",
+ "pos_x": -18,
+ "pos_y": -24.15625,
+ "pos_z": 81.96875,
+ "stations": "Hermite Horizons"
+ },
+ {
+ "name": "Grabil",
+ "pos_x": -2.96875,
+ "pos_y": -1.46875,
+ "pos_z": 127.4375,
+ "stations": "Bailey Laboratory,Klimuk Terminal,Cadamosto Survey"
+ },
+ {
+ "name": "Grabrakan",
+ "pos_x": 157.84375,
+ "pos_y": -163.34375,
+ "pos_z": -40.0625,
+ "stations": "Chacornac Dock,Skjellerup Vision,Brendan Horizons"
+ },
+ {
+ "name": "Grabri",
+ "pos_x": -97.71875,
+ "pos_y": 43.65625,
+ "pos_z": -15.78125,
+ "stations": "Hamilton Hub,Pierce Legacy,Matteucci Prospect"
+ },
+ {
+ "name": "Grabrigpa",
+ "pos_x": -26.0625,
+ "pos_y": 0.375,
+ "pos_z": 89.25,
+ "stations": "Sternberg Ring,Qian Port"
+ },
+ {
+ "name": "Grabru",
+ "pos_x": -95.15625,
+ "pos_y": 117.625,
+ "pos_z": 40.75,
+ "stations": "Henize Platform,Springer Terminal"
+ },
+ {
+ "name": "Grafias",
+ "pos_x": 37.6875,
+ "pos_y": 0.5,
+ "pos_z": 126.8125,
+ "stations": "Gubarev Landing,Fraley Ring,Plexico Market,Barnaby Forum"
+ },
+ {
+ "name": "Graill Redd",
+ "pos_x": -4.5625,
+ "pos_y": 75.03125,
+ "pos_z": -40.15625,
+ "stations": "Womack Orbital,J. G. Ballard Gateway,Czerneda Port,Ray Dock,Czerneda Gateway,Frobenius Legacy"
+ },
+ {
+ "name": "Gram",
+ "pos_x": -95.5,
+ "pos_y": -25.53125,
+ "pos_z": -30.65625,
+ "stations": "Thorne Landing,Hernandez's Claim"
+ },
+ {
+ "name": "Gran",
+ "pos_x": 86.5625,
+ "pos_y": 59.8125,
+ "pos_z": 0.59375,
+ "stations": "Flade Prospect,Friend Barracks,Balandin Horizons"
+ },
+ {
+ "name": "Grana",
+ "pos_x": 73.40625,
+ "pos_y": -102.21875,
+ "pos_z": 118.0625,
+ "stations": "Oikawa Asylum,Pilyugin Outpost"
+ },
+ {
+ "name": "Grandmort",
+ "pos_x": 134.71875,
+ "pos_y": 13.71875,
+ "pos_z": 88.34375,
+ "stations": "Ziljak Prospect"
+ },
+ {
+ "name": "Grans",
+ "pos_x": 141.53125,
+ "pos_y": 23.9375,
+ "pos_z": 85.15625,
+ "stations": "Viehbock Landing"
+ },
+ {
+ "name": "Granthaimi",
+ "pos_x": -52.71875,
+ "pos_y": 24.125,
+ "pos_z": 41.09375,
+ "stations": "Parmitano Colony"
+ },
+ {
+ "name": "Graudika",
+ "pos_x": 57.46875,
+ "pos_y": -79.53125,
+ "pos_z": 60.90625,
+ "stations": "Gustafsson Terminal,Kludze Keep,Kidd Plant"
+ },
+ {
+ "name": "Graudung",
+ "pos_x": 5.09375,
+ "pos_y": -90.90625,
+ "pos_z": 135.625,
+ "stations": "Ohain Mines,Baily Platform,Shimizu Platform,Malaspina Landing,Kandel Barracks"
+ },
+ {
+ "name": "Graudus",
+ "pos_x": -51.625,
+ "pos_y": -143.375,
+ "pos_z": 55.21875,
+ "stations": "Skripochka Dock,Garn Installation,Vela Station,Filipchenko Terminal,Lee's Folly"
+ },
+ {
+ "name": "Grauie",
+ "pos_x": -26.21875,
+ "pos_y": -55.25,
+ "pos_z": 128.53125,
+ "stations": "Metcalf Refinery"
+ },
+ {
+ "name": "Graung",
+ "pos_x": 176.6875,
+ "pos_y": -129.40625,
+ "pos_z": -11.25,
+ "stations": "Coggia Vision,van Royen Port,Arzachel Ring,Hadamard Point"
+ },
+ {
+ "name": "Grealli",
+ "pos_x": 174.9375,
+ "pos_y": -220.1875,
+ "pos_z": 16.59375,
+ "stations": "Medupe Settlement"
+ },
+ {
+ "name": "Greallu",
+ "pos_x": 102.75,
+ "pos_y": 45.34375,
+ "pos_z": -108.125,
+ "stations": "Burton Stop,Stafford Keep,Back's Folly"
+ },
+ {
+ "name": "Grebatabea",
+ "pos_x": -75.96875,
+ "pos_y": -121.40625,
+ "pos_z": -62.65625,
+ "stations": "Akers Enterprise,Hausdorff Arsenal,Obruchev Prospect"
+ },
+ {
+ "name": "Grebato",
+ "pos_x": 136.46875,
+ "pos_y": -23.09375,
+ "pos_z": 67.125,
+ "stations": "Williams Relay"
+ },
+ {
+ "name": "Grebayeb",
+ "pos_x": 20.125,
+ "pos_y": -2.71875,
+ "pos_z": -152.875,
+ "stations": "Gurney Relay"
+ },
+ {
+ "name": "Grebegus",
+ "pos_x": 125.65625,
+ "pos_y": -177.53125,
+ "pos_z": 53.15625,
+ "stations": "Thomas Orbital,Kondo Port,Kanai Vision,Banneker Vision,Aoki Orbital,Wolszczan Vision,Holdstock Beacon,Adragna Orbital,Potocnik Dock,Hughes Base,Sei Terminal,Staden's Pride,Vogel's Inheritance,Leberecht Tempel Landing,Mitchison Arsenal"
+ },
+ {
+ "name": "Grebinawair",
+ "pos_x": 108.0625,
+ "pos_y": -74.84375,
+ "pos_z": 95.3125,
+ "stations": "Emshwiller Colony"
+ },
+ {
+ "name": "Grebo",
+ "pos_x": 61.15625,
+ "pos_y": 89.5,
+ "pos_z": 103.25,
+ "stations": "Gottlob Frege Dock,McCandless Relay,Payson Silo"
+ },
+ {
+ "name": "Gree",
+ "pos_x": -36.5,
+ "pos_y": 65.15625,
+ "pos_z": -45.59375,
+ "stations": "Hopkinson Port,Wnuk-Lipinski's Progress,Coulter Hub,Tennyson d'Eyncourt Depot"
+ },
+ {
+ "name": "Greidmarang",
+ "pos_x": 104.59375,
+ "pos_y": 37.375,
+ "pos_z": 132.375,
+ "stations": "Tucker Prospect"
+ },
+ {
+ "name": "Greidu",
+ "pos_x": 97.96875,
+ "pos_y": -196.25,
+ "pos_z": -14.65625,
+ "stations": "Auzout Orbital,Wafa Vision,Hynek Gateway,Wales Depot,MacGill Prospect"
+ },
+ {
+ "name": "Greila",
+ "pos_x": -5.90625,
+ "pos_y": 35.375,
+ "pos_z": 63.90625,
+ "stations": "Smith Terminal,Oltion Hub,Leavitt Horizons"
+ },
+ {
+ "name": "Greklis",
+ "pos_x": 15.21875,
+ "pos_y": -90.96875,
+ "pos_z": 118.21875,
+ "stations": "Lee City"
+ },
+ {
+ "name": "Grendel",
+ "pos_x": 84.46875,
+ "pos_y": 2.6875,
+ "pos_z": -29.96875,
+ "stations": "Skvortsov Dock,Sharma Works,Lerman Arsenal"
+ },
+ {
+ "name": "Grid",
+ "pos_x": -163.1875,
+ "pos_y": -24.5,
+ "pos_z": -85.5625,
+ "stations": "Lloyd Dock"
+ },
+ {
+ "name": "Gridge",
+ "pos_x": 64.71875,
+ "pos_y": -118.25,
+ "pos_z": 101.25,
+ "stations": "Salpeter Terminal,Sitterly Orbital,Unsold Hub,Backlund Gateway,Ohain Orbital,Evans Hub,Roelofs Orbital,Chiang Orbital,Grimwood Camp,Tago Barracks,Metz Installation,Britnev's Inheritance,Maury Camp"
+ },
+ {
+ "name": "Gridii",
+ "pos_x": -80.15625,
+ "pos_y": -91.9375,
+ "pos_z": -19.34375,
+ "stations": "Xing Horizons"
+ },
+ {
+ "name": "Gridiuhet",
+ "pos_x": -139.375,
+ "pos_y": -73.78125,
+ "pos_z": 80.4375,
+ "stations": "Macgregor Dock,Galindo Station,Butcher Holdings,Conti Works"
+ },
+ {
+ "name": "Groa",
+ "pos_x": 126,
+ "pos_y": -183.03125,
+ "pos_z": -59.03125,
+ "stations": "Thierree Prospect,Wallerstein Terminal,Mooz Barracks,Davidson Prospect"
+ },
+ {
+ "name": "Groanomana",
+ "pos_x": 102.46875,
+ "pos_y": -49.09375,
+ "pos_z": 6.875,
+ "stations": "Naylor Enterprise,Harrison Dock"
+ },
+ {
+ "name": "Groombridge 1618",
+ "pos_x": -2.34375,
+ "pos_y": 12.625,
+ "pos_z": -9.34375,
+ "stations": "Gernsback Terminal,Cady Market,Franklin Ring,Martinez Market,Szilard Arsenal,Mouhot Prospect,Quimper Lab,George's Pride"
+ },
+ {
+ "name": "Groombridge 34",
+ "pos_x": -9.90625,
+ "pos_y": -3.6875,
+ "pos_z": -5.09375,
+ "stations": "Matthews City,Jones Estate,Ford City,McCandless Colony,Sawyer's Pride"
+ },
+ {
+ "name": "Grovicele",
+ "pos_x": 163.34375,
+ "pos_y": 21.03125,
+ "pos_z": 21,
+ "stations": "Yamazaki Station,McNair Dock,Pike Observatory,Beltrami Landing"
+ },
+ {
+ "name": "Grovichan",
+ "pos_x": 115.4375,
+ "pos_y": 39.59375,
+ "pos_z": -81.03125,
+ "stations": "Shiras Reformatory"
+ },
+ {
+ "name": "Grovicho",
+ "pos_x": 114.5625,
+ "pos_y": -174.90625,
+ "pos_z": -52.78125,
+ "stations": "Marsden Port,Hill Tinsley Terminal,Kerimov Camp,Cardano's Progress"
+ },
+ {
+ "name": "Grovichun",
+ "pos_x": 72.90625,
+ "pos_y": -112.375,
+ "pos_z": 59.46875,
+ "stations": "Yerka City"
+ },
+ {
+ "name": "Grovii",
+ "pos_x": 96.59375,
+ "pos_y": 42,
+ "pos_z": -52.84375,
+ "stations": "Filipchenko Hub,Wescott Ring,Bondar Gateway,Volk Terminal,Haarsma Port,Fidalgo Point,Bell Station,Forbes Vista,Gell-Mann Enterprise,Fairbairn Station,White Horizons"
+ },
+ {
+ "name": "Grovoy",
+ "pos_x": 162.875,
+ "pos_y": -181.375,
+ "pos_z": 36.90625,
+ "stations": "Kapteyn Platform"
+ },
+ {
+ "name": "Gru Hypue KS-T d3-31",
+ "pos_x": -4990.84375,
+ "pos_y": -935.71875,
+ "pos_z": 13387.15625,
+ "stations": "Gagarin Gate"
+ },
+ {
+ "name": "Grud",
+ "pos_x": 191.75,
+ "pos_y": -149.4375,
+ "pos_z": 66.8125,
+ "stations": "Gonnessiat Outpost"
+ },
+ {
+ "name": "Grudevaci",
+ "pos_x": 22.40625,
+ "pos_y": -77.96875,
+ "pos_z": -29.71875,
+ "stations": "Archimedes Outpost"
+ },
+ {
+ "name": "Grudi",
+ "pos_x": 112,
+ "pos_y": -11.71875,
+ "pos_z": -14.6875,
+ "stations": "Burke Market,Moriarty Hub,Cremona Orbital,Matthews Port,Lee Terminal,Heinlein Port"
+ },
+ {
+ "name": "Grudii",
+ "pos_x": 79.6875,
+ "pos_y": -155.875,
+ "pos_z": 79.75,
+ "stations": "Parsons Relay"
+ },
+ {
+ "name": "GT Pegasi",
+ "pos_x": -45.46875,
+ "pos_y": -20.90625,
+ "pos_z": -4.125,
+ "stations": "Bella Terminal,Foreman Settlement"
+ },
+ {
+ "name": "Guai",
+ "pos_x": 155.8125,
+ "pos_y": -114.875,
+ "pos_z": 47.9375,
+ "stations": "Ferguson Station,Froud Prospect,Pond Base"
+ },
+ {
+ "name": "Guai Shou",
+ "pos_x": 73.0625,
+ "pos_y": -51.875,
+ "pos_z": -102.03125,
+ "stations": "Bakewell Gateway,Bykovsky Hub,Rushworth Port,Baker Depot"
+ },
+ {
+ "name": "Guambia",
+ "pos_x": 19,
+ "pos_y": 19.59375,
+ "pos_z": -133.125,
+ "stations": "Eddington Platform,Baturin Colony,Geston Works"
+ },
+ {
+ "name": "Guamni",
+ "pos_x": -52.40625,
+ "pos_y": 12.0625,
+ "pos_z": 122.875,
+ "stations": "Powell Penal colony,Chargaff Enterprise"
+ },
+ {
+ "name": "Guan",
+ "pos_x": -40.75,
+ "pos_y": 82.71875,
+ "pos_z": 78.59375,
+ "stations": "Aksyonov Hub"
+ },
+ {
+ "name": "Guan Dun",
+ "pos_x": 49,
+ "pos_y": -105.40625,
+ "pos_z": 121.375,
+ "stations": "Bouwens Orbital,Royo Station,Brunner Ring,Watts Mine"
+ },
+ {
+ "name": "Guan Gong",
+ "pos_x": 138.4375,
+ "pos_y": 60.8125,
+ "pos_z": -41.125,
+ "stations": "Marley Base,Farouk Beacon"
+ },
+ {
+ "name": "Guan Tun",
+ "pos_x": 15.5,
+ "pos_y": -60.125,
+ "pos_z": 127.1875,
+ "stations": "Teng-hui Dock,Crouch Orbital,Hartlib Settlement,Turzillo Hub,Farouk Refinery"
+ },
+ {
+ "name": "Guan Wango",
+ "pos_x": 40.9375,
+ "pos_y": -180.65625,
+ "pos_z": -1.84375,
+ "stations": "Paczynski Enterprise,Maupertuis Terminal,Brill Orbital,Fallows Terminal,Jones Landing,Bolton Camp,Bainbridge Silo"
+ },
+ {
+ "name": "Guan Yun",
+ "pos_x": 94.375,
+ "pos_y": -104.78125,
+ "pos_z": -34.3125,
+ "stations": "Darlton Terminal,Wylie Dock,Gibson Dock,Jones Installation,Walters' Claim"
+ },
+ {
+ "name": "Guan Zaihe",
+ "pos_x": -53.4375,
+ "pos_y": -39.6875,
+ "pos_z": -125.5,
+ "stations": "Hammond Gateway"
+ },
+ {
+ "name": "Guan Zana",
+ "pos_x": -131.6875,
+ "pos_y": 119.75,
+ "pos_z": 112.84375,
+ "stations": "Brule Orbital,Kurland Enterprise"
+ },
+ {
+ "name": "Guana Bhun",
+ "pos_x": 208.625,
+ "pos_y": -127,
+ "pos_z": 20.4375,
+ "stations": "Hertzsprung Mine,Miller Survey,Penrose Reach"
+ },
+ {
+ "name": "Guanangu",
+ "pos_x": 99.28125,
+ "pos_y": -130.21875,
+ "pos_z": 37.96875,
+ "stations": "Sylvester Lab,Young Platform,Richer Station"
+ },
+ {
+ "name": "Guanatamas",
+ "pos_x": -52.34375,
+ "pos_y": 40.3125,
+ "pos_z": -114.4375,
+ "stations": "Morin Point"
+ },
+ {
+ "name": "Guanekagha",
+ "pos_x": -144.4375,
+ "pos_y": 48.21875,
+ "pos_z": 10.9375,
+ "stations": "Drebbel Station,Leckie's Progress,Skvortsov Holdings"
+ },
+ {
+ "name": "Guaneponne",
+ "pos_x": 58,
+ "pos_y": -118.34375,
+ "pos_z": 77.78125,
+ "stations": "Kapteyn Vision,Zoline Horizons,Robert Colony"
+ },
+ {
+ "name": "Guanggi",
+ "pos_x": 18.84375,
+ "pos_y": 113.5625,
+ "pos_z": -53.15625,
+ "stations": "Moriarty Gateway,Drzewiecki Depot,Palmer Ring,Hendel Port,Norman's Folly"
+ },
+ {
+ "name": "Guangguba",
+ "pos_x": -125.15625,
+ "pos_y": -80.96875,
+ "pos_z": -81.1875,
+ "stations": "Tiptree Settlement,Adams Vision,Tanaka Enterprise"
+ },
+ {
+ "name": "Guangngel",
+ "pos_x": 159.9375,
+ "pos_y": -82.40625,
+ "pos_z": 75.84375,
+ "stations": "Kaluta Dock,Vuia Port,Sandage Terminal,Keeler Plant"
+ },
+ {
+ "name": "Guangul",
+ "pos_x": -27.5,
+ "pos_y": 14.3125,
+ "pos_z": -2.28125,
+ "stations": "Blaha Station,Poleshchuk Terminal,Cockrell Survey"
+ },
+ {
+ "name": "Guanomo",
+ "pos_x": 24,
+ "pos_y": -101.65625,
+ "pos_z": -142.84375,
+ "stations": "Shalatula Outpost"
+ },
+ {
+ "name": "Guarada",
+ "pos_x": -109.09375,
+ "pos_y": -2.53125,
+ "pos_z": 39.65625,
+ "stations": "Borel Outpost,Mattingly Beacon"
+ },
+ {
+ "name": "Guarakma",
+ "pos_x": 98.59375,
+ "pos_y": -32.125,
+ "pos_z": -102.125,
+ "stations": "Yegorov Dock,Onnes Mine,Murphy Hub"
+ },
+ {
+ "name": "Guaras",
+ "pos_x": -89.65625,
+ "pos_y": -0.1875,
+ "pos_z": 2.5,
+ "stations": "Hawley Port,Klimuk Ring,Doi Colony,Morgan Penal colony"
+ },
+ {
+ "name": "Guari",
+ "pos_x": -36,
+ "pos_y": -24.46875,
+ "pos_z": -136.28125,
+ "stations": "Vlamingh Port"
+ },
+ {
+ "name": "Guarijio",
+ "pos_x": 51.03125,
+ "pos_y": -238.78125,
+ "pos_z": 22.0625,
+ "stations": "Arend Stop"
+ },
+ {
+ "name": "Guarpulici",
+ "pos_x": 120.8125,
+ "pos_y": -68.625,
+ "pos_z": 52.8125,
+ "stations": "Goldschmidt Terminal,De Relay,Reeves Refinery"
+ },
+ {
+ "name": "Guatae",
+ "pos_x": 26.5625,
+ "pos_y": 102.40625,
+ "pos_z": -127.21875,
+ "stations": "Eschbach Hub"
+ },
+ {
+ "name": "Guathiti",
+ "pos_x": 93.90625,
+ "pos_y": -94.15625,
+ "pos_z": -15.09375,
+ "stations": "Messier Port,Suntzeff Station,Wenzel Port,Atwood Works,Houtman Landing"
+ },
+ {
+ "name": "Guayambaan",
+ "pos_x": -132,
+ "pos_y": 0.78125,
+ "pos_z": -14.0625,
+ "stations": "Willis Station,Laumer Station,Jones Ring,Chun Orbital,Galouye Hub,Wylie Bastion,Zetford Holdings,Kozin Dock,Gann Terminal,Fast City,Mallory Holdings,Payne Station,Mitchell City"
+ },
+ {
+ "name": "Guayana",
+ "pos_x": 142.25,
+ "pos_y": 7.03125,
+ "pos_z": 54.90625,
+ "stations": "Fossey Orbital,Humboldt Depot"
+ },
+ {
+ "name": "Guaycurua",
+ "pos_x": 103.59375,
+ "pos_y": -2.4375,
+ "pos_z": -135.15625,
+ "stations": "Hedley Dock,Aguirre Bastion"
+ },
+ {
+ "name": "Guayeb",
+ "pos_x": -118.78125,
+ "pos_y": -3.25,
+ "pos_z": 32.5625,
+ "stations": "Phillpotts Prospect,Hendel Terminal,Barr Installation"
+ },
+ {
+ "name": "Gubia",
+ "pos_x": 107.375,
+ "pos_y": 76.375,
+ "pos_z": 76.46875,
+ "stations": "Konscak Ring,Doctorow Settlement,Noli Bastion,Fernao do Po Orbital"
+ },
+ {
+ "name": "Gubiakum",
+ "pos_x": -82.875,
+ "pos_y": -16.75,
+ "pos_z": -61.375,
+ "stations": "Boulle Dock,Keyes Plant"
+ },
+ {
+ "name": "Gubian",
+ "pos_x": 76.625,
+ "pos_y": -98.21875,
+ "pos_z": 4.125,
+ "stations": "Crossfield Terminal,Townes Landing"
+ },
+ {
+ "name": "Gucub Baja",
+ "pos_x": -34.25,
+ "pos_y": 76.59375,
+ "pos_z": 98.90625,
+ "stations": "Van Scyoc Settlement,Stross Vision"
+ },
+ {
+ "name": "Gucuma",
+ "pos_x": -73.3125,
+ "pos_y": -80.375,
+ "pos_z": -114.03125,
+ "stations": "Brule Gateway,Morris Laboratory,Effinger Ring,Bugrov Installation"
+ },
+ {
+ "name": "Gucumadhyas",
+ "pos_x": -89.6875,
+ "pos_y": -97.09375,
+ "pos_z": -76.875,
+ "stations": "Cenker City,Sagan Landing,Bethe Installation,Galindo Point,Rennie Station"
+ },
+ {
+ "name": "Gucuman",
+ "pos_x": 111.625,
+ "pos_y": 0.15625,
+ "pos_z": 105.125,
+ "stations": "Teller Orbital,Chun Observatory,Britnev Barracks"
+ },
+ {
+ "name": "Gucumatassa",
+ "pos_x": -43.0625,
+ "pos_y": -151.59375,
+ "pos_z": -1.34375,
+ "stations": "Kojima Landing,von Biela Port"
+ },
+ {
+ "name": "Gucumatz",
+ "pos_x": 0.5,
+ "pos_y": -180.3125,
+ "pos_z": 19.71875,
+ "stations": "Abell Relay,Luyten Beacon"
+ },
+ {
+ "name": "Gudramo",
+ "pos_x": 30.6875,
+ "pos_y": 14.15625,
+ "pos_z": 92.46875,
+ "stations": "Bolotov Mines,Peterson Colony"
+ },
+ {
+ "name": "Gudran",
+ "pos_x": 70.40625,
+ "pos_y": -47.96875,
+ "pos_z": -94.75,
+ "stations": "Fuglesang's Progress,Bakewell Survey"
+ },
+ {
+ "name": "Gudu",
+ "pos_x": -114.9375,
+ "pos_y": 57.3125,
+ "pos_z": 6.25,
+ "stations": "Aguirre Enterprise"
+ },
+ {
+ "name": "Gudus",
+ "pos_x": 115.59375,
+ "pos_y": -78.84375,
+ "pos_z": -50.3125,
+ "stations": "Kulin's Claim"
+ },
+ {
+ "name": "Guede",
+ "pos_x": -97.0625,
+ "pos_y": -67.6875,
+ "pos_z": 4.375,
+ "stations": "Wiener Settlement"
+ },
+ {
+ "name": "Guei",
+ "pos_x": 9.375,
+ "pos_y": 19.28125,
+ "pos_z": -97,
+ "stations": "Creighton Mines,Precourt Platform,Elcano Town,Mohmand Dock"
+ },
+ {
+ "name": "Guei Goab",
+ "pos_x": 31.90625,
+ "pos_y": -9.40625,
+ "pos_z": 174.78125,
+ "stations": "Ballard Arena"
+ },
+ {
+ "name": "Guerre",
+ "pos_x": 72.3125,
+ "pos_y": -104.53125,
+ "pos_z": -93.46875,
+ "stations": "Schmidt Prospect,Kosai Enterprise,Pond Station"
+ },
+ {
+ "name": "Guerrongal",
+ "pos_x": -56,
+ "pos_y": -105.09375,
+ "pos_z": 82.96875,
+ "stations": "Zillig Platform,White City,Barnwell Enterprise,Schweinfurth Horizons"
+ },
+ {
+ "name": "Gugu",
+ "pos_x": -77.4375,
+ "pos_y": 91.5,
+ "pos_z": -61.21875,
+ "stations": "Corte-Real Dock"
+ },
+ {
+ "name": "Gugulukunn",
+ "pos_x": -56.125,
+ "pos_y": 145.03125,
+ "pos_z": 85.40625,
+ "stations": "Nasmyth Works"
+ },
+ {
+ "name": "Guguroro",
+ "pos_x": -18.84375,
+ "pos_y": -119.34375,
+ "pos_z": 42.90625,
+ "stations": "Goodricke Enterprise,Trujillo Vision,Zeppelin Dock"
+ },
+ {
+ "name": "Gugurun",
+ "pos_x": 101.09375,
+ "pos_y": -61.03125,
+ "pos_z": 41.46875,
+ "stations": "Stephan Terminal,Farghani Station,Moore Station,Yamamoto Hub,Cesar Janssen Terminal,Bushkov Depot"
+ },
+ {
+ "name": "Guguurtuu",
+ "pos_x": -77.5,
+ "pos_y": 50,
+ "pos_z": 65.65625,
+ "stations": "Roberts Enterprise"
+ },
+ {
+ "name": "Guguynich",
+ "pos_x": 3.1875,
+ "pos_y": -154.125,
+ "pos_z": 43.15625,
+ "stations": "Arisman Beacon"
+ },
+ {
+ "name": "Gui Banni",
+ "pos_x": -104.875,
+ "pos_y": 81.65625,
+ "pos_z": -27.90625,
+ "stations": "Wilder Port,Cowper Penal colony,Ocampo Gateway,Archer Observatory"
+ },
+ {
+ "name": "Gui Hulha",
+ "pos_x": 26.28125,
+ "pos_y": -28.4375,
+ "pos_z": -95.53125,
+ "stations": "Gilliland Legacy,Erdos Arsenal"
+ },
+ {
+ "name": "Gui Renes",
+ "pos_x": 51.0625,
+ "pos_y": -21.84375,
+ "pos_z": 110.40625,
+ "stations": "Hopkins Port,Donaldson Barracks"
+ },
+ {
+ "name": "Gui Wande",
+ "pos_x": -129.78125,
+ "pos_y": 4.28125,
+ "pos_z": -7.6875,
+ "stations": "Clement Station,Aikin Horizons,Vinge Base,Andrews Terminal,Jeury's Progress"
+ },
+ {
+ "name": "Gui Xian",
+ "pos_x": 145.28125,
+ "pos_y": -120.15625,
+ "pos_z": -2.03125,
+ "stations": "Pritchard Orbital,Bartini Port"
+ },
+ {
+ "name": "Guie",
+ "pos_x": 157.8125,
+ "pos_y": -128.59375,
+ "pos_z": -41.125,
+ "stations": "Hollander Reach,Howe's Exile,Alvarez de Pineda Landing"
+ },
+ {
+ "name": "Guinasan",
+ "pos_x": -39.84375,
+ "pos_y": -253.625,
+ "pos_z": 98.6875,
+ "stations": "Aller Landing"
+ },
+ {
+ "name": "Guincans",
+ "pos_x": -93.71875,
+ "pos_y": 45.53125,
+ "pos_z": 77.875,
+ "stations": "Brust Dock,Brunel Horizons"
+ },
+ {
+ "name": "Guinna",
+ "pos_x": 216.71875,
+ "pos_y": -131,
+ "pos_z": 61.78125,
+ "stations": "Dyr Relay"
+ },
+ {
+ "name": "Guitaiku",
+ "pos_x": -49.84375,
+ "pos_y": -13.5625,
+ "pos_z": -95.4375,
+ "stations": "Shosuke Mine"
+ },
+ {
+ "name": "Guite",
+ "pos_x": -57.1875,
+ "pos_y": -87.375,
+ "pos_z": -57.03125,
+ "stations": "Harris' Progress,Chadwick Orbital,Ampere Holdings,Binet Orbital"
+ },
+ {
+ "name": "Gulians",
+ "pos_x": 24.09375,
+ "pos_y": 107.8125,
+ "pos_z": -34.9375,
+ "stations": "Pangborn Platform"
+ },
+ {
+ "name": "Gulidjan",
+ "pos_x": -10.96875,
+ "pos_y": -109.0625,
+ "pos_z": -11.5,
+ "stations": "Smith Plant,Alvares Plant,Kandel Refinery"
+ },
+ {
+ "name": "Guligali",
+ "pos_x": -88.71875,
+ "pos_y": 11.96875,
+ "pos_z": 169.84375,
+ "stations": "Gaspar de Portola Mines,Bates Terminal,Reis Depot"
+ },
+ {
+ "name": "Gulis",
+ "pos_x": 94.03125,
+ "pos_y": -24.90625,
+ "pos_z": -115.78125,
+ "stations": "Wang Beacon"
+ },
+ {
+ "name": "Gullapites",
+ "pos_x": 75.15625,
+ "pos_y": -51.15625,
+ "pos_z": -26.03125,
+ "stations": "Noguchi Station,Hoften Survey,Garnier Prison,Garn Relay"
+ },
+ {
+ "name": "Gullici",
+ "pos_x": 96.8125,
+ "pos_y": -44.875,
+ "pos_z": 55.03125,
+ "stations": "Hardy Lab,Melvin Port"
+ },
+ {
+ "name": "Gullveti",
+ "pos_x": -119.84375,
+ "pos_y": -21.0625,
+ "pos_z": -116.15625,
+ "stations": "Sargent Gateway,Binder Terminal,Moran Colony,Tall Landing,Fidalgo Enterprise"
+ },
+ {
+ "name": "Gulngaba",
+ "pos_x": 61.59375,
+ "pos_y": 9.09375,
+ "pos_z": 92.1875,
+ "stations": "Ford Port,Galiano Orbital,Kent Ring,Galindo Dock,Norton Enterprise,Bisson Hub,Rawat Port,Abasheli Prospect,Watts' Inheritance"
+ },
+ {
+ "name": "Gulngatha",
+ "pos_x": 122.875,
+ "pos_y": -182.65625,
+ "pos_z": -5.875,
+ "stations": "Mandel Station,Gunter Colony,Dittmar Base"
+ },
+ {
+ "name": "Gun",
+ "pos_x": -13.8125,
+ "pos_y": -42.625,
+ "pos_z": 7.96875,
+ "stations": "Gotlieb Refinery,Sleator Prospect,Garnier Point"
+ },
+ {
+ "name": "Gunab",
+ "pos_x": -100.1875,
+ "pos_y": -104.4375,
+ "pos_z": -83.875,
+ "stations": "Ozanne Dock,Szentmartony Enterprise"
+ },
+ {
+ "name": "Gunai",
+ "pos_x": 156.25,
+ "pos_y": 44.375,
+ "pos_z": -54.75,
+ "stations": "Sylvester Point,Oramus Ring,Converse Vision"
+ },
+ {
+ "name": "Gunama",
+ "pos_x": -121.875,
+ "pos_y": -40.09375,
+ "pos_z": 27.28125,
+ "stations": "Levi-Civita Installation,Bose Installation"
+ },
+ {
+ "name": "Gunapa",
+ "pos_x": 8.96875,
+ "pos_y": -98.375,
+ "pos_z": -81.96875,
+ "stations": "Haber Orbital,Yaping Port,Galton Enterprise,Kwolek Keep,Stott Town,Gibson Vision"
+ },
+ {
+ "name": "Gunapai",
+ "pos_x": 69.03125,
+ "pos_y": -50.8125,
+ "pos_z": 117.65625,
+ "stations": "Behring Works,Collins Installation,Crown Beacon"
+ },
+ {
+ "name": "Gunapisni",
+ "pos_x": -55.9375,
+ "pos_y": -6.96875,
+ "pos_z": 85.9375,
+ "stations": "Descartes Station,Lem Relay,Ehrlich Station"
+ },
+ {
+ "name": "Gunapiti",
+ "pos_x": 44.03125,
+ "pos_y": -61.8125,
+ "pos_z": 60.71875,
+ "stations": "Shinjo Terminal,Fox Orbital,Tanner Keep"
+ },
+ {
+ "name": "Gunavian",
+ "pos_x": 2.46875,
+ "pos_y": -121.21875,
+ "pos_z": 120.28125,
+ "stations": "Filippenko Port,Fox Port,Marriott Dock,O'Neill Holdings,Lefschetz City,Akers Bastion"
+ },
+ {
+ "name": "Gunavidji",
+ "pos_x": 57.4375,
+ "pos_y": 106.25,
+ "pos_z": -10.46875,
+ "stations": "Satcher Prospect"
+ },
+ {
+ "name": "Gunawa",
+ "pos_x": 103.40625,
+ "pos_y": 83.46875,
+ "pos_z": -8.3125,
+ "stations": "Archambault City,Chang-Diaz Ring"
+ },
+ {
+ "name": "Gunayeb",
+ "pos_x": -16.1875,
+ "pos_y": -125.78125,
+ "pos_z": 93.65625,
+ "stations": "Mohr Terminal,Oterma Dock,Kier Enterprise,Martins Depot,Struve Enterprise"
+ },
+ {
+ "name": "Gundiae",
+ "pos_x": -129.40625,
+ "pos_y": 98.34375,
+ "pos_z": 30.9375,
+ "stations": "Bernard Colony"
+ },
+ {
+ "name": "Gundji",
+ "pos_x": 43.8125,
+ "pos_y": -249.65625,
+ "pos_z": 36.75,
+ "stations": "Goryu Orbital,Sheepshanks Platform,J. G. Ballard Hub,Harrison Dock"
+ },
+ {
+ "name": "Gungamato",
+ "pos_x": 106.1875,
+ "pos_y": 7.6875,
+ "pos_z": -105.375,
+ "stations": "Brera Hub,Zelazny Gateway,Due Dock,Maury Refinery,Moore Exchange,Mallory Vision"
+ },
+ {
+ "name": "Gungassa",
+ "pos_x": -15.0625,
+ "pos_y": 123.96875,
+ "pos_z": -105.375,
+ "stations": "Borisenko Dock"
+ },
+ {
+ "name": "Gungkuni",
+ "pos_x": 99.90625,
+ "pos_y": -22.71875,
+ "pos_z": -46.21875,
+ "stations": "Sevastyanov Dock"
+ },
+ {
+ "name": "Gungnir",
+ "pos_x": -9.6875,
+ "pos_y": 15.9375,
+ "pos_z": 102.28125,
+ "stations": "Meucci Port,Aleksandrov Vision"
+ },
+ {
+ "name": "Gungo",
+ "pos_x": 5.3125,
+ "pos_y": -177.625,
+ "pos_z": -13.96875,
+ "stations": "Tombaugh Depot,Ohain Hub,Kibalchich Hangar"
+ },
+ {
+ "name": "Gungu",
+ "pos_x": -129.40625,
+ "pos_y": -85.875,
+ "pos_z": -69.3125,
+ "stations": "Altman Depot"
+ },
+ {
+ "name": "Gunnlodumla",
+ "pos_x": -14.25,
+ "pos_y": 175.03125,
+ "pos_z": -77.21875,
+ "stations": "Bunch Mine"
+ },
+ {
+ "name": "Gunno",
+ "pos_x": 79.6875,
+ "pos_y": -21.03125,
+ "pos_z": 100,
+ "stations": "Pierce Survey,Zewail Settlement,Nowak Works"
+ },
+ {
+ "name": "Gunnodayak",
+ "pos_x": 138.78125,
+ "pos_y": -101.96875,
+ "pos_z": 23.34375,
+ "stations": "Brundage Gateway,Alexeyev Beacon,Meinel Port,Pu Hub,Mies van der Rohe Plant,Chaviano Enterprise"
+ },
+ {
+ "name": "Gunnovale",
+ "pos_x": -8.03125,
+ "pos_y": 14.15625,
+ "pos_z": 64.46875,
+ "stations": "Bradley Station,Clauss City,Gentle Hub"
+ },
+ {
+ "name": "Gunnovii",
+ "pos_x": -114.40625,
+ "pos_y": 37.25,
+ "pos_z": -1.71875,
+ "stations": "Vaez de Torres Refinery,Reilly Hangar,Gernhardt Base"
+ },
+ {
+ "name": "Gunnunggu",
+ "pos_x": 130.96875,
+ "pos_y": -21.78125,
+ "pos_z": 114,
+ "stations": "Windt Colony,Yourkevitch Settlement"
+ },
+ {
+ "name": "Gunnus",
+ "pos_x": 27.96875,
+ "pos_y": -21.28125,
+ "pos_z": -131,
+ "stations": "Tucker Colony,Roddenberry Platform,Dayuan Hub,Russell Relay,Lorenz Penal colony"
+ },
+ {
+ "name": "Gunwi",
+ "pos_x": 156.375,
+ "pos_y": -19.6875,
+ "pos_z": 35.375,
+ "stations": "Mayer Camp"
+ },
+ {
+ "name": "Gunwin",
+ "pos_x": 82.28125,
+ "pos_y": -100.71875,
+ "pos_z": 131.1875,
+ "stations": "Sugie Terminal,McKee Ring,Niven Bastion,Ford Lab"
+ },
+ {
+ "name": "Gunwingaa",
+ "pos_x": -98.46875,
+ "pos_y": 26.21875,
+ "pos_z": -19.8125,
+ "stations": "Laumer Plant"
+ },
+ {
+ "name": "Gunwinggu",
+ "pos_x": -20.6875,
+ "pos_y": -140.625,
+ "pos_z": 65.09375,
+ "stations": "Youll Horizons,Lichtenberg Stop,Brundage Survey"
+ },
+ {
+ "name": "Gunwini",
+ "pos_x": 83.125,
+ "pos_y": 21,
+ "pos_z": -83.4375,
+ "stations": "Whitney Lab,Tanaka Refinery"
+ },
+ {
+ "name": "Gunwites",
+ "pos_x": 87.75,
+ "pos_y": -135.84375,
+ "pos_z": -85.3125,
+ "stations": "Ilyushin Platform,al-Din al-Urdi Port,Kettle Arsenal"
+ },
+ {
+ "name": "Gunwithhogg",
+ "pos_x": -106.78125,
+ "pos_y": -2.375,
+ "pos_z": -68.46875,
+ "stations": "Wiener Estate"
+ },
+ {
+ "name": "Guo Chima",
+ "pos_x": -130.21875,
+ "pos_y": 62.34375,
+ "pos_z": -11.25,
+ "stations": "Ross Orbital,May Bastion,LeConte Base,Legendre Dock"
+ },
+ {
+ "name": "Guo Zi",
+ "pos_x": 117.71875,
+ "pos_y": 20.28125,
+ "pos_z": 40.9375,
+ "stations": "Crook Base,Wells Legacy"
+ },
+ {
+ "name": "Guoji",
+ "pos_x": -64.71875,
+ "pos_y": -88.8125,
+ "pos_z": 36.71875,
+ "stations": "Kirchoff Terminal,Mukai Horizons,Pasteur Landing,Nakaya Bastion"
+ },
+ {
+ "name": "Gura",
+ "pos_x": 120.03125,
+ "pos_y": -135.5,
+ "pos_z": 15.84375,
+ "stations": "Alden Terminal,Dreyer Vision,Ziewe Penal colony"
+ },
+ {
+ "name": "Gurabru",
+ "pos_x": 3.59375,
+ "pos_y": 15.34375,
+ "pos_z": -126.46875,
+ "stations": "Kazantsev Orbital,Thesiger Port,Kratman Hub,Plexico Dock,Hui Ring,Ferguson Enterprise,Kurland City,Wollheim Survey,Nylund Survey"
+ },
+ {
+ "name": "Guracana",
+ "pos_x": 29.84375,
+ "pos_y": 118.78125,
+ "pos_z": 9.65625,
+ "stations": "Williamson Vision,Gwynn Survey"
+ },
+ {
+ "name": "Guragwe",
+ "pos_x": -26.9375,
+ "pos_y": 56.25,
+ "pos_z": 58.0625,
+ "stations": "Otiman Gateway,Onizuka Ring"
+ },
+ {
+ "name": "Gurney Slade",
+ "pos_x": 70.21875,
+ "pos_y": 53.28125,
+ "pos_z": 81,
+ "stations": "Adkins Terminal,Diamond Landing,Bushkov Oasis"
+ },
+ {
+ "name": "Gurojas",
+ "pos_x": 179,
+ "pos_y": -164.21875,
+ "pos_z": 87.8125,
+ "stations": "Hariot Survey"
+ },
+ {
+ "name": "Guroji",
+ "pos_x": -98.3125,
+ "pos_y": -13.53125,
+ "pos_z": -99.96875,
+ "stations": "Conti Mines,Effinger Horizons,Teng Plant"
+ },
+ {
+ "name": "Gurua",
+ "pos_x": 120.78125,
+ "pos_y": -196.125,
+ "pos_z": -42.6875,
+ "stations": "Suydam Vision,Westerhout Terminal,Pinto Prospect,Friedman Relay"
+ },
+ {
+ "name": "Gurughna",
+ "pos_x": 97.3125,
+ "pos_y": 42.375,
+ "pos_z": 39.59375,
+ "stations": "Tiptree Dock,Great Platform"
+ },
+ {
+ "name": "Guruku Yi",
+ "pos_x": -76.5,
+ "pos_y": -79.46875,
+ "pos_z": -38.0625,
+ "stations": "Feynman Dock"
+ },
+ {
+ "name": "Gurumukas",
+ "pos_x": 59,
+ "pos_y": 55.9375,
+ "pos_z": -70.9375,
+ "stations": "Tryggvason Refinery,Simonyi Platform"
+ },
+ {
+ "name": "Gurus",
+ "pos_x": 113.28125,
+ "pos_y": 51.6875,
+ "pos_z": -57.59375,
+ "stations": "Daley Terminal,Kingsmill Orbital,Dantec Hub,Hartlib Arsenal"
+ },
+ {
+ "name": "Guttond",
+ "pos_x": 134.90625,
+ "pos_y": -177.6875,
+ "pos_z": 106,
+ "stations": "Neumann Mine"
+ },
+ {
+ "name": "Guttors",
+ "pos_x": 37.28125,
+ "pos_y": -154.3125,
+ "pos_z": -20.25,
+ "stations": "Mrkos Terminal,Tietjen City,Pajdusakova Port,Dalmas Stop,Cottenot Gateway,McHugh Arsenal"
+ },
+ {
+ "name": "Guttun",
+ "pos_x": 22.84375,
+ "pos_y": -18.09375,
+ "pos_z": 86.78125,
+ "stations": "Grover Terminal,Schottky Gateway,Bohm Settlement,Chorel Legacy"
+ },
+ {
+ "name": "Guttunthur",
+ "pos_x": -57.03125,
+ "pos_y": 84,
+ "pos_z": -91.3125,
+ "stations": "Vetulani Vision"
+ },
+ {
+ "name": "Guugupala",
+ "pos_x": 185.5,
+ "pos_y": -178.21875,
+ "pos_z": 20.8125,
+ "stations": "Veron Base,Burbidge's Claim"
+ },
+ {
+ "name": "Guuguyni",
+ "pos_x": 130.84375,
+ "pos_y": -96.46875,
+ "pos_z": 67.5625,
+ "stations": "Shen Terminal,Zaschka Depot,Weill Ring,Puiseux Terminal"
+ },
+ {
+ "name": "Guy",
+ "pos_x": 26.71875,
+ "pos_y": 14.25,
+ "pos_z": 7.375,
+ "stations": "Georg Bothe Port,Leclerc Dock,Mondeh Terminal,Lavoisier Installation,McCandless Terminal,Stafford Dock,Clapperton Landing,Keyes Enterprise,Qurra Orbital,Goeschke Enterprise,Rolland Relay"
+ },
+ {
+ "name": "Gwaelod",
+ "pos_x": 26.6875,
+ "pos_y": -21.40625,
+ "pos_z": -2.6875,
+ "stations": "Veach Gateway,von Helmont Terminal,Rothman Terminal,Wnuk-Lipinski Plant,Meikle Hub"
+ },
+ {
+ "name": "Gwion",
+ "pos_x": 145.34375,
+ "pos_y": -32.5625,
+ "pos_z": 45.375,
+ "stations": "Zhigang Colony"
+ },
+ {
+ "name": "Gwiona",
+ "pos_x": 14.4375,
+ "pos_y": 157.5,
+ "pos_z": -64.15625,
+ "stations": "Gibson Station,Waldeck Orbital,Langford Terminal,Cantor Depot,Kratman Base,Lee Enterprise"
+ },
+ {
+ "name": "Gwiones",
+ "pos_x": -39.625,
+ "pos_y": -77.96875,
+ "pos_z": 50.03125,
+ "stations": "Miklouho-Maclay Colony,Tudela's Inheritance,Fuchs Terminal,Bloomfield's Claim"
+ },
+ {
+ "name": "Gwri",
+ "pos_x": -32.625,
+ "pos_y": -51.84375,
+ "pos_z": 111.5625,
+ "stations": "Bloch Port,Poindexter Landing,Illy Port,Newton Platform,Wnuk-Lipinski Beacon"
+ },
+ {
+ "name": "Gwydans",
+ "pos_x": -134.28125,
+ "pos_y": -20.28125,
+ "pos_z": 5.625,
+ "stations": "Remec Hub,Gerlache Orbital,Vries Dock,Lasswitz Settlement,Oramus Holdings,Clifton Dock,Lewitt Vision,Hippalus Relay,Hutchinson Landing,Sinclair Depot"
+ },
+ {
+ "name": "Gwyddi",
+ "pos_x": 82.03125,
+ "pos_y": -142.40625,
+ "pos_z": 96.9375,
+ "stations": "Hoffleit Enterprise,Pollas Installation,Shaw Survey,Karman Orbital"
+ },
+ {
+ "name": "Gwyddi Di",
+ "pos_x": 180.65625,
+ "pos_y": -133.84375,
+ "pos_z": 47.09375,
+ "stations": "Pickering Depot"
+ },
+ {
+ "name": "Gwyddioneni",
+ "pos_x": -59.65625,
+ "pos_y": 66.1875,
+ "pos_z": -45.9375,
+ "stations": "Napier Prospect,Moore Hub,Nobel Holdings"
+ },
+ {
+ "name": "GY Bootis",
+ "pos_x": -16.1875,
+ "pos_y": 102.5625,
+ "pos_z": 31.8125,
+ "stations": "Savinykh Horizons,Noriega Terminal"
+ },
+ {
+ "name": "Gyhldekala",
+ "pos_x": -3.21875,
+ "pos_y": -19.59375,
+ "pos_z": 142.65625,
+ "stations": "Filter Survey"
+ },
+ {
+ "name": "Gyhldemal",
+ "pos_x": 128.34375,
+ "pos_y": -165.0625,
+ "pos_z": -37.84375,
+ "stations": "Barr Dock,Hollander Platform"
+ },
+ {
+ "name": "Gyhlder",
+ "pos_x": 141.34375,
+ "pos_y": -201.65625,
+ "pos_z": 119.90625,
+ "stations": "Foglio Depot"
+ },
+ {
+ "name": "Gyton's Hope",
+ "pos_x": -29.625,
+ "pos_y": -2,
+ "pos_z": -26.75,
+ "stations": "Margulies Hub,Gyton's Eyrie,Kennan Depot,Iskareen"
+ },
+ {
+ "name": "Gyvata",
+ "pos_x": 40.59375,
+ "pos_y": 71.875,
+ "pos_z": -124.9375,
+ "stations": "Conklin Platform,North Port,Gardner Horizons"
+ },
+ {
+ "name": "Gyvata Indi",
+ "pos_x": 77.59375,
+ "pos_y": -35.46875,
+ "pos_z": 9.34375,
+ "stations": "Phillpotts Base"
+ },
+ {
+ "name": "Gyvatanoy",
+ "pos_x": -102.625,
+ "pos_y": 18.03125,
+ "pos_z": 80.875,
+ "stations": "Borlaug Dock"
+ },
+ {
+ "name": "Gyvates",
+ "pos_x": -111.875,
+ "pos_y": -74.59375,
+ "pos_z": -33.71875,
+ "stations": "Wright Port,Thoreau Mines"
+ },
+ {
+ "name": "Gyvathaime",
+ "pos_x": 138.5625,
+ "pos_y": -183.5625,
+ "pos_z": 17.625,
+ "stations": "Marcy Dock,de Kamp Enterprise"
+ },
+ {
+ "name": "Gyvati",
+ "pos_x": 15.5625,
+ "pos_y": 36.6875,
+ "pos_z": 60.625,
+ "stations": "Brillant Base,Pryor Landing,Skolem Hangar,Berners-Lee Laboratory"
+ },
+ {
+ "name": "Gyvatices",
+ "pos_x": 101.78125,
+ "pos_y": -126.40625,
+ "pos_z": -12.78125,
+ "stations": "Walter Dock,Couper Gateway"
+ },
+ {
+ "name": "Gyvatiges",
+ "pos_x": 23.25,
+ "pos_y": 2.28125,
+ "pos_z": 78.59375,
+ "stations": "Stackpole Keep,Vaucanson City,Patsayev Enterprise"
+ },
+ {
+ "name": "h Draconis",
+ "pos_x": -39.84375,
+ "pos_y": 29.5625,
+ "pos_z": -3.90625,
+ "stations": "Janjetov Memorial,Brislington,Erika's Rest,Chorel Enterprise"
+ },
+ {
+ "name": "h Eridani",
+ "pos_x": 97,
+ "pos_y": -145.875,
+ "pos_z": -57.21875,
+ "stations": "Juan de la Cierva Penal colony,Bahcall's Folly"
+ },
+ {
+ "name": "H Puppis",
+ "pos_x": 184.8125,
+ "pos_y": -61.84375,
+ "pos_z": -32.40625,
+ "stations": "Matheson Dock,Cook Settlement,Jones Dock,Shinn Dock"
+ },
+ {
+ "name": "Hach",
+ "pos_x": -73.0625,
+ "pos_y": 13.28125,
+ "pos_z": -8.25,
+ "stations": "Zudov Orbital,Evans City,Macdonald's Folly,Galilei Station,Foale Works"
+ },
+ {
+ "name": "Hachan Ku",
+ "pos_x": -154.9375,
+ "pos_y": 10.3125,
+ "pos_z": 41.0625,
+ "stations": "Giles Terminal,Houtman Prospect"
+ },
+ {
+ "name": "Hachihikaru",
+ "pos_x": -17.5,
+ "pos_y": 63.625,
+ "pos_z": 61.75,
+ "stations": "Varthema Dock,Simmons Gateway,Clifton Ring,Cartmill Ring,Maury Silo,Popper Works,Gottlob Frege Enterprise"
+ },
+ {
+ "name": "Hachiman",
+ "pos_x": -72.1875,
+ "pos_y": -19.84375,
+ "pos_z": 118.3125,
+ "stations": "Fabian Market"
+ },
+ {
+ "name": "Hachimr",
+ "pos_x": 44.5625,
+ "pos_y": 66.34375,
+ "pos_z": -130.625,
+ "stations": "Bennett Settlement,Oersted Station,Slade Lab"
+ },
+ {
+ "name": "Hadad",
+ "pos_x": 9.875,
+ "pos_y": 57.84375,
+ "pos_z": 20.59375,
+ "stations": "Baker Point,Baird Installation,Windt Mine"
+ },
+ {
+ "name": "Haeduwong",
+ "pos_x": 58.8125,
+ "pos_y": 66.4375,
+ "pos_z": 65.625,
+ "stations": "Banks Depot,Bulleid Settlement,Tombaugh Colony,Whitworth Hub"
+ },
+ {
+ "name": "Hagalaz",
+ "pos_x": -50.59375,
+ "pos_y": 45.15625,
+ "pos_z": 11.09375,
+ "stations": "Russell Hub,Henslow Dock,Piccard Enterprise,Laird Landing,Anderson Settlement"
+ },
+ {
+ "name": "Haghole",
+ "pos_x": -5.875,
+ "pos_y": 0.90625,
+ "pos_z": 23.84375,
+ "stations": "Messerschmid City,Block Base"
+ },
+ {
+ "name": "Hagr",
+ "pos_x": 41.65625,
+ "pos_y": 0.0625,
+ "pos_z": 83.625,
+ "stations": "Green Base,Sharman Plant"
+ },
+ {
+ "name": "Hahgwe",
+ "pos_x": 20.53125,
+ "pos_y": 7.375,
+ "pos_z": -60.90625,
+ "stations": "Skripochka Horizons,Barcelos Camp,Hertz Colony"
+ },
+ {
+ "name": "Hai Chan",
+ "pos_x": 74.59375,
+ "pos_y": 88.75,
+ "pos_z": 120.25,
+ "stations": "Sladek Ring,Gann Base,McMahon Ring"
+ },
+ {
+ "name": "Hai Ho",
+ "pos_x": -2.59375,
+ "pos_y": -43.28125,
+ "pos_z": -42.21875,
+ "stations": "Feynman Orbital,Dobrovolskiy Vista"
+ },
+ {
+ "name": "Hai Norsh",
+ "pos_x": 86.1875,
+ "pos_y": 19.375,
+ "pos_z": -51.4375,
+ "stations": "Kimberlin Port,Kube-McDowell Orbital,Tiedemann Port,Rasch Hub,Stillman Lab"
+ },
+ {
+ "name": "Hai Si",
+ "pos_x": -147.46875,
+ "pos_y": -48.5,
+ "pos_z": 32.3125,
+ "stations": "Tanner Mines,Wilder Prospect,Tem Depot"
+ },
+ {
+ "name": "Haiden",
+ "pos_x": -142.03125,
+ "pos_y": -13.34375,
+ "pos_z": -43.90625,
+ "stations": "Searfoss Enterprise,Desargues Depot"
+ },
+ {
+ "name": "Haidjaram",
+ "pos_x": -126.3125,
+ "pos_y": 57.28125,
+ "pos_z": -24.1875,
+ "stations": "Laval Orbital,Borlaug Station,Voss Gateway,Garay Port,Brunel Beacon,Lu Ring,Remek City,VanderMeer Landing,Carson Relay"
+ },
+ {
+ "name": "Hais",
+ "pos_x": -81.71875,
+ "pos_y": 2.375,
+ "pos_z": -141.8125,
+ "stations": "Back Beacon,Quaglia Works"
+ },
+ {
+ "name": "Haisienet",
+ "pos_x": 101.3125,
+ "pos_y": -35.5625,
+ "pos_z": 96.75,
+ "stations": "Porubcan Dock,Jefferies Beacon,Sharp Barracks"
+ },
+ {
+ "name": "Haita",
+ "pos_x": 0.625,
+ "pos_y": -4.40625,
+ "pos_z": 135.03125,
+ "stations": "MacCurdy Settlement"
+ },
+ {
+ "name": "Haitchane",
+ "pos_x": -80.3125,
+ "pos_y": -148.8125,
+ "pos_z": -80.53125,
+ "stations": "Thompson Landing,Chalker Installation,Bretnor Port"
+ },
+ {
+ "name": "Haithis",
+ "pos_x": 5.34375,
+ "pos_y": 29.75,
+ "pos_z": 112.59375,
+ "stations": "Andersson Relay,Fraas Gateway,Ramaswamy Settlement,Crippen Port,Nelder Relay"
+ },
+ {
+ "name": "Haiurici",
+ "pos_x": -130.5,
+ "pos_y": -22.28125,
+ "pos_z": 0.90625,
+ "stations": "Midgeley Installation,Fermat Silo"
+ },
+ {
+ "name": "Hajand",
+ "pos_x": -141.59375,
+ "pos_y": -26.53125,
+ "pos_z": 37.46875,
+ "stations": "Hahn Stop,Ingstad Retreat"
+ },
+ {
+ "name": "Hajangai",
+ "pos_x": -28.125,
+ "pos_y": 4.90625,
+ "pos_z": -68.28125,
+ "stations": "Lee Orbital,Schwann Port,Prunariu Survey,Ibold Enterprise,Hambly Arena"
+ },
+ {
+ "name": "Hajangerni",
+ "pos_x": -132.8125,
+ "pos_y": 15.65625,
+ "pos_z": -47.90625,
+ "stations": "Diesel Stop,Kovalyonok Mine,Baker Refinery"
+ },
+ {
+ "name": "Hajanheimr",
+ "pos_x": 59.75,
+ "pos_y": 54.125,
+ "pos_z": 73.5625,
+ "stations": "Koch Base,Patsayev Base,Griggs Horizons"
+ },
+ {
+ "name": "Hajani",
+ "pos_x": -106.65625,
+ "pos_y": -35.03125,
+ "pos_z": -148.28125,
+ "stations": "Evans Orbital"
+ },
+ {
+ "name": "Hajanisei",
+ "pos_x": 108.71875,
+ "pos_y": -251.9375,
+ "pos_z": 43.5625,
+ "stations": "Morrill Platform,Dassault Mines"
+ },
+ {
+ "name": "Hajapai",
+ "pos_x": 0.65625,
+ "pos_y": 20.5,
+ "pos_z": -164.46875,
+ "stations": "Bulleid Terminal,Al-Battani Dock,Griffith Point,Shaara Relay"
+ },
+ {
+ "name": "Hajara",
+ "pos_x": 53.75,
+ "pos_y": -183.5,
+ "pos_z": -21.78125,
+ "stations": "Sitterly Platform"
+ },
+ {
+ "name": "Hajarage",
+ "pos_x": 42.84375,
+ "pos_y": 151.90625,
+ "pos_z": 37.0625,
+ "stations": "Petaja Horizons,Rey Orbital,Zetford Hub,Kerr's Progress"
+ },
+ {
+ "name": "Hajauja",
+ "pos_x": 148.71875,
+ "pos_y": 53.375,
+ "pos_z": -5.9375,
+ "stations": "de Sousa Mine,Okorafor Point"
+ },
+ {
+ "name": "Hajo",
+ "pos_x": -28.5,
+ "pos_y": -0.0625,
+ "pos_z": 85.53125,
+ "stations": "Darwin Orbital,Georg Bothe Port"
+ },
+ {
+ "name": "Hajon",
+ "pos_x": 127.78125,
+ "pos_y": -148.8125,
+ "pos_z": -59.375,
+ "stations": "Heiles Dock,Bruck Prospect,Bertin Relay"
+ },
+ {
+ "name": "Hajong",
+ "pos_x": 48.9375,
+ "pos_y": 9.65625,
+ "pos_z": -127,
+ "stations": "Crampton Dock,Carrier Dock,Liebig Station,Sarich Laboratory"
+ },
+ {
+ "name": "Hajonga",
+ "pos_x": 91.375,
+ "pos_y": -129.71875,
+ "pos_z": 5.9375,
+ "stations": "Flammarion Hub"
+ },
+ {
+ "name": "Hakili",
+ "pos_x": 39.03125,
+ "pos_y": -31.46875,
+ "pos_z": -41.15625,
+ "stations": "Koch Hub,Wescott City,Ellis Settlement"
+ },
+ {
+ "name": "Hakkaia",
+ "pos_x": 104.96875,
+ "pos_y": -12.6875,
+ "pos_z": -34.25,
+ "stations": "Johnson Gateway"
+ },
+ {
+ "name": "Hakkinoha",
+ "pos_x": -33.75,
+ "pos_y": -45.78125,
+ "pos_z": -65.34375,
+ "stations": "Siegel Base,Lawhead Survey,Garnier Prospect"
+ },
+ {
+ "name": "Hakkipikki",
+ "pos_x": -53.5,
+ "pos_y": 77.8125,
+ "pos_z": 8.9375,
+ "stations": "Laing's Folly,de Balboa Stop"
+ },
+ {
+ "name": "Hakkipikun",
+ "pos_x": 1.5625,
+ "pos_y": 128.84375,
+ "pos_z": -43.625,
+ "stations": "Gibbs' Pride,Egan Dock"
+ },
+ {
+ "name": "Halai",
+ "pos_x": -63.5,
+ "pos_y": 24.65625,
+ "pos_z": 32.375,
+ "stations": "Chaudhary Enterprise,Cenker Station,Bartoe Terminal,al-Khayyam Enterprise"
+ },
+ {
+ "name": "Halamaitu",
+ "pos_x": 125.96875,
+ "pos_y": -104.5625,
+ "pos_z": 28.03125,
+ "stations": "Harding Base"
+ },
+ {
+ "name": "Halanduni",
+ "pos_x": 164.5625,
+ "pos_y": -218.28125,
+ "pos_z": 37.0625,
+ "stations": "Celsius Station,Bahcall Orbital,Furuta Beacon"
+ },
+ {
+ "name": "Halaye",
+ "pos_x": -46.15625,
+ "pos_y": -54.75,
+ "pos_z": 76.1875,
+ "stations": "Savinykh Orbital,McClintock Port,Curbeam Base,Werner von Siemens Dock,Chretien Observatory"
+ },
+ {
+ "name": "Halbangaay",
+ "pos_x": -51.9375,
+ "pos_y": -95.625,
+ "pos_z": 10.0625,
+ "stations": "Haack Gateway,Hill Tinsley Orbital,Valigursky Station,Charlois Orbital,Drake Port"
+ },
+ {
+ "name": "Halbara",
+ "pos_x": 131.78125,
+ "pos_y": -108.46875,
+ "pos_z": 107.96875,
+ "stations": "Wasden Colony,Kidinnu City,Busemann Station,Gonnessiat Point,Lee Relay"
+ },
+ {
+ "name": "Halbarapii",
+ "pos_x": -158.46875,
+ "pos_y": 19.34375,
+ "pos_z": -22.34375,
+ "stations": "Shiner Station,Fidalgo Port,Low Barracks,Andersson Hub,Avogadro Horizons"
+ },
+ {
+ "name": "Hamal",
+ "pos_x": -30.5,
+ "pos_y": -38.46875,
+ "pos_z": -43.875,
+ "stations": "Hermaszewski Dock,Poincare Point"
+ },
+ {
+ "name": "Hambing",
+ "pos_x": 44.4375,
+ "pos_y": -63.46875,
+ "pos_z": 118.9375,
+ "stations": "Hirayama Holdings,Green Plant,Goldschmidt Holdings,Flettner Dock,Drake Hub"
+ },
+ {
+ "name": "Hambo",
+ "pos_x": -110.8125,
+ "pos_y": -12.15625,
+ "pos_z": -100.53125,
+ "stations": "Fernandes de Queiros Survey,Dana Beacon"
+ },
+ {
+ "name": "Hambula",
+ "pos_x": -39.65625,
+ "pos_y": 6.125,
+ "pos_z": -2.5,
+ "stations": "Payne Horizons,Piccard Gateway,Malaspina Bastion,Lind Base"
+ },
+ {
+ "name": "Hamlet's Harmony",
+ "pos_x": -9549.4375,
+ "pos_y": -909.1875,
+ "pos_z": 19795.875,
+ "stations": "McDonald Platform"
+ },
+ {
+ "name": "Hamoco",
+ "pos_x": -128.03125,
+ "pos_y": 57.46875,
+ "pos_z": 85.75,
+ "stations": "Broglie Enterprise,Tani Survey,Noguchi Settlement,Kummer's Progress"
+ },
+ {
+ "name": "Han Crua",
+ "pos_x": 145.375,
+ "pos_y": -51.15625,
+ "pos_z": 93.40625,
+ "stations": "Ayton Innes Terminal,Kreutz Keep"
+ },
+ {
+ "name": "Han Tsanyan",
+ "pos_x": 129.9375,
+ "pos_y": -235.6875,
+ "pos_z": 29.90625,
+ "stations": "Giunta Vision"
+ },
+ {
+ "name": "Han Wa",
+ "pos_x": -28.75,
+ "pos_y": -112.21875,
+ "pos_z": -54.25,
+ "stations": "Armstrong Platform,Dzhanibekov Port"
+ },
+ {
+ "name": "Han Xiang",
+ "pos_x": 90.8125,
+ "pos_y": -79.25,
+ "pos_z": 6.34375,
+ "stations": "Thome Hub"
+ },
+ {
+ "name": "Hang Po",
+ "pos_x": 56.5,
+ "pos_y": -81.84375,
+ "pos_z": -22.84375,
+ "stations": "Marshburn Hub,Ericsson Dock,Miletus Point,Serebrov Port,Shaara Prospect"
+ },
+ {
+ "name": "Hanggardi",
+ "pos_x": -19.03125,
+ "pos_y": 7.375,
+ "pos_z": 56.5,
+ "stations": "Soddy Market,Stott City,Henricks Gateway"
+ },
+ {
+ "name": "Hangkan",
+ "pos_x": 91.5625,
+ "pos_y": -204.21875,
+ "pos_z": 100.34375,
+ "stations": "Hirn Port,Townes Barracks"
+ },
+ {
+ "name": "Hangu",
+ "pos_x": -40.53125,
+ "pos_y": -141.78125,
+ "pos_z": 8.75,
+ "stations": "Bova Settlement,Dias' Pride"
+ },
+ {
+ "name": "Hani",
+ "pos_x": 65.6875,
+ "pos_y": 79.21875,
+ "pos_z": -36.46875,
+ "stations": "Payne Orbital,Swanwick Depot"
+ },
+ {
+ "name": "Hania",
+ "pos_x": 105.25,
+ "pos_y": -222.59375,
+ "pos_z": 71.46875,
+ "stations": "Fallows Vision,Hawker Holdings,Pacheco Terminal,Wells Bastion"
+ },
+ {
+ "name": "Hanissi",
+ "pos_x": 78.28125,
+ "pos_y": -217.03125,
+ "pos_z": -42,
+ "stations": "Gentil Prospect,Cartier Vision,Flaugergues Prospect"
+ },
+ {
+ "name": "Hanneng",
+ "pos_x": -119.46875,
+ "pos_y": 45.0625,
+ "pos_z": 0.0625,
+ "stations": "Baudin Gateway,Tavernier Station,Bugrov Depot,MacLeod Orbital,Kessel Enterprise,Lopez de Legazpi Enterprise"
+ },
+ {
+ "name": "Hannheir",
+ "pos_x": 67.75,
+ "pos_y": -163.03125,
+ "pos_z": 101.65625,
+ "stations": "Walotsky Depot,Ahnert-Rohlfs Landing"
+ },
+ {
+ "name": "Hannonner",
+ "pos_x": 145.65625,
+ "pos_y": -90.96875,
+ "pos_z": -19.96875,
+ "stations": "Lorrah Settlement,Warner Dock,McDaniel Camp"
+ },
+ {
+ "name": "Hannos",
+ "pos_x": -62.34375,
+ "pos_y": -121.21875,
+ "pos_z": -60.46875,
+ "stations": "Tryggvason Mine"
+ },
+ {
+ "name": "Hanu",
+ "pos_x": 1.15625,
+ "pos_y": 84.25,
+ "pos_z": 139.625,
+ "stations": "Savery Terminal,Wrangel Silo"
+ },
+ {
+ "name": "Hanuku",
+ "pos_x": -105.5625,
+ "pos_y": 110.28125,
+ "pos_z": 7.28125,
+ "stations": "Whymper Gateway,Fawcett Landing,Kolmogorov Port,Bradley Arsenal,Evans Depot"
+ },
+ {
+ "name": "Hanukuntit",
+ "pos_x": 38.53125,
+ "pos_y": -213.8125,
+ "pos_z": 48.46875,
+ "stations": "Maunder Platform,Sudworth Mines"
+ },
+ {
+ "name": "Hanung Tzu",
+ "pos_x": -28.53125,
+ "pos_y": -44.6875,
+ "pos_z": 11.125,
+ "stations": "Fraley Vision,Behnken Penal colony"
+ },
+ {
+ "name": "Haokah",
+ "pos_x": -22.1875,
+ "pos_y": 37.625,
+ "pos_z": -47.3125,
+ "stations": "Revin Horizons,Tito Landing,Stiegler Prospect"
+ },
+ {
+ "name": "Haoki",
+ "pos_x": 7.75,
+ "pos_y": 59,
+ "pos_z": 120.46875,
+ "stations": "Saberhagen Holdings"
+ },
+ {
+ "name": "Haokipi",
+ "pos_x": 35,
+ "pos_y": -93.71875,
+ "pos_z": -69.6875,
+ "stations": "Ikeya Station,Selous Relay"
+ },
+ {
+ "name": "Haokit",
+ "pos_x": 26.4375,
+ "pos_y": 49.15625,
+ "pos_z": -96.3125,
+ "stations": "Sagan Settlement,Divis Base,Carlisle Reach"
+ },
+ {
+ "name": "Haoko",
+ "pos_x": 100.5625,
+ "pos_y": -181.78125,
+ "pos_z": 31.4375,
+ "stations": "Ptack Relay"
+ },
+ {
+ "name": "Haolin Ti",
+ "pos_x": 88.4375,
+ "pos_y": -19.5,
+ "pos_z": 92.3125,
+ "stations": "Acaba Beacon,Fuglesang Survey"
+ },
+ {
+ "name": "Haoria",
+ "pos_x": -4.5,
+ "pos_y": -104.125,
+ "pos_z": 52.875,
+ "stations": "Suydam Survey,Muramatsu Works"
+ },
+ {
+ "name": "Hap",
+ "pos_x": 159.28125,
+ "pos_y": -169.28125,
+ "pos_z": 20.75,
+ "stations": "Witt Station,Israel Landing,Eddington Bastion,Chongzhi Survey"
+ },
+ {
+ "name": "Hapakhered",
+ "pos_x": 127.15625,
+ "pos_y": -23.09375,
+ "pos_z": -67.09375,
+ "stations": "Liwei Terminal,Dobrovolski Colony,Franklin Station,Skripochka Prospect,Quaglia Plant"
+ },
+ {
+ "name": "Hapats",
+ "pos_x": -13.40625,
+ "pos_y": -21.21875,
+ "pos_z": 150.65625,
+ "stations": "Hudson Depot,Chaviano Dock"
+ },
+ {
+ "name": "Har",
+ "pos_x": -50.0625,
+ "pos_y": -40.625,
+ "pos_z": 43.15625,
+ "stations": "Kopra Holdings"
+ },
+ {
+ "name": "Har Ibofan",
+ "pos_x": 116.90625,
+ "pos_y": -164.15625,
+ "pos_z": -16.625,
+ "stations": "Rogers Station,Mueller City,Laming Enterprise,Jones Landing"
+ },
+ {
+ "name": "Har Ibora",
+ "pos_x": -119.0625,
+ "pos_y": -52.9375,
+ "pos_z": -64,
+ "stations": "Herjulfsson Refinery"
+ },
+ {
+ "name": "Har Ibori",
+ "pos_x": 135.25,
+ "pos_y": -36.8125,
+ "pos_z": -36.34375,
+ "stations": "Holden Platform"
+ },
+ {
+ "name": "Har Itari",
+ "pos_x": 52.8125,
+ "pos_y": 37.4375,
+ "pos_z": 56.9375,
+ "stations": "Garneau Hub,Anders Orbital,Currie Orbital,Rzeppa Orbital,Mohri Terminal,Galindo Settlement,Harper Landing"
+ },
+ {
+ "name": "Har Itaris",
+ "pos_x": -19.34375,
+ "pos_y": 129.3125,
+ "pos_z": -104.0625,
+ "stations": "Nicollet Arena"
+ },
+ {
+ "name": "Har Itariu",
+ "pos_x": -111.09375,
+ "pos_y": 10.875,
+ "pos_z": -6.8125,
+ "stations": "Grandin City,Ivanov Vision,Hadfield Prospect,Hodgson's Inheritance"
+ },
+ {
+ "name": "Har Ne Zha",
+ "pos_x": 100.96875,
+ "pos_y": -69.28125,
+ "pos_z": 106.46875,
+ "stations": "Tsunenaga's Claim,Lynden-Bell Outpost"
+ },
+ {
+ "name": "Har Pa Chiu",
+ "pos_x": -109.875,
+ "pos_y": 101.03125,
+ "pos_z": 56.84375,
+ "stations": "Dukaj Vision"
+ },
+ {
+ "name": "Har Pa Hsin",
+ "pos_x": -6.3125,
+ "pos_y": 125.46875,
+ "pos_z": -35.03125,
+ "stations": "Manning City,Benyovszky Landing,Simak Settlement"
+ },
+ {
+ "name": "Har Pa Kams",
+ "pos_x": 165.6875,
+ "pos_y": -192.8125,
+ "pos_z": 2.5625,
+ "stations": "Lyot Port,Chertovsky Platform,Dall Installation"
+ },
+ {
+ "name": "Har Pahary",
+ "pos_x": 21.625,
+ "pos_y": -92.8125,
+ "pos_z": -43.09375,
+ "stations": "Leonard Station,Lindemann Hub,Anderson Settlement,Ziewe Vision"
+ },
+ {
+ "name": "Harada",
+ "pos_x": -112.25,
+ "pos_y": 19.09375,
+ "pos_z": 107.65625,
+ "stations": "Dobzhansky Dock,Artyukhin Mines"
+ },
+ {
+ "name": "Haradhyas",
+ "pos_x": 106.1875,
+ "pos_y": 15.21875,
+ "pos_z": -99.46875,
+ "stations": "Cardano Plant,Norton Beacon"
+ },
+ {
+ "name": "Harai",
+ "pos_x": -59.46875,
+ "pos_y": 16,
+ "pos_z": 140.59375,
+ "stations": "Celebi Prospect,Derleth Settlement,Clough Works"
+ },
+ {
+ "name": "Haraka",
+ "pos_x": 5.875,
+ "pos_y": 16.4375,
+ "pos_z": -90.21875,
+ "stations": "Bulgakov Installation,Lem Dock,Blackman Station"
+ },
+ {
+ "name": "Harakwaxaca",
+ "pos_x": 60.40625,
+ "pos_y": 40.03125,
+ "pos_z": -94.53125,
+ "stations": "Williams Survey,Selberg's Pride,Higginbotham's Inheritance"
+ },
+ {
+ "name": "Harang",
+ "pos_x": -114.84375,
+ "pos_y": -59.96875,
+ "pos_z": -79.375,
+ "stations": "Brera Base,Gerrold Vision,Francisco de Almeida Vision"
+ },
+ {
+ "name": "Haras",
+ "pos_x": -154.40625,
+ "pos_y": -10.0625,
+ "pos_z": 3.4375,
+ "stations": "al-Khayyam Outpost"
+ },
+ {
+ "name": "Hardalsumi",
+ "pos_x": 188.3125,
+ "pos_y": -143.625,
+ "pos_z": -16.75,
+ "stations": "Huxley Relay,Carpenter Colony,Dufay Terminal"
+ },
+ {
+ "name": "Hardh",
+ "pos_x": -53.59375,
+ "pos_y": -103.96875,
+ "pos_z": 29.90625,
+ "stations": "Hadfield Dock,Soddy Dock"
+ },
+ {
+ "name": "Hardovit",
+ "pos_x": 137.65625,
+ "pos_y": -177.96875,
+ "pos_z": 6,
+ "stations": "Bennett Platform"
+ },
+ {
+ "name": "Hared",
+ "pos_x": -97.125,
+ "pos_y": 22.75,
+ "pos_z": -20,
+ "stations": "Sekowski Dock,Poisson Settlement,Allen Base"
+ },
+ {
+ "name": "Hareg",
+ "pos_x": -91.875,
+ "pos_y": -56.15625,
+ "pos_z": -149.4375,
+ "stations": "Steinmuller's Inheritance,Cadamosto Settlement,Leichhardt Base"
+ },
+ {
+ "name": "Haren",
+ "pos_x": 124.5,
+ "pos_y": 113.40625,
+ "pos_z": -50.4375,
+ "stations": "Leichhardt Gateway,Wisniewski-Snerg Dock,Velho Arsenal"
+ },
+ {
+ "name": "Harermid",
+ "pos_x": -82.40625,
+ "pos_y": -18.875,
+ "pos_z": 53.09375,
+ "stations": "Pausch Plant,Henslow Barracks,Evans Hub"
+ },
+ {
+ "name": "Harete",
+ "pos_x": -131.5625,
+ "pos_y": 11.25,
+ "pos_z": 1.03125,
+ "stations": "Derleth Colony"
+ },
+ {
+ "name": "Hariates",
+ "pos_x": 70.15625,
+ "pos_y": -72.625,
+ "pos_z": -71.78125,
+ "stations": "Fuglesang Port,Cseszneky Port,Fuglesang Hub,Cassidy Plant,Alexeyev Relay"
+ },
+ {
+ "name": "Harich",
+ "pos_x": -125.59375,
+ "pos_y": 91.0625,
+ "pos_z": -64.96875,
+ "stations": "Marlowe Depot,Brillant Settlement"
+ },
+ {
+ "name": "Harigah",
+ "pos_x": 166.3125,
+ "pos_y": -136.1875,
+ "pos_z": 102.125,
+ "stations": "Bereznyak Station,Constantine Enterprise,Vakhmistrov Landing,Okuni Terminal,Desargues Depot"
+ },
+ {
+ "name": "Harihas",
+ "pos_x": 17.65625,
+ "pos_y": 85.375,
+ "pos_z": 142.90625,
+ "stations": "Zahn Plant,Godwin Plant,Okorafor Enterprise"
+ },
+ {
+ "name": "Harima",
+ "pos_x": 44.46875,
+ "pos_y": 26.875,
+ "pos_z": -110.5625,
+ "stations": "Nourse Point,Gilliland Survey,Schirra Holdings"
+ },
+ {
+ "name": "Haritana",
+ "pos_x": 46.15625,
+ "pos_y": 49.34375,
+ "pos_z": 57.5625,
+ "stations": "Lorrah Station,Yano Terminal,Keyes' Folly,Byrd Dock"
+ },
+ {
+ "name": "Haritanis",
+ "pos_x": -64.5625,
+ "pos_y": -89.625,
+ "pos_z": -70.65625,
+ "stations": "Bottego Gateway,Evans Terminal,Gentle Gateway,Shatner Port,Sheffield Station"
+ },
+ {
+ "name": "Harites",
+ "pos_x": -85.21875,
+ "pos_y": -134.125,
+ "pos_z": -143,
+ "stations": "Doyle Port,Shiner Horizons"
+ },
+ {
+ "name": "Hariti",
+ "pos_x": -55.125,
+ "pos_y": 1.5625,
+ "pos_z": 85.40625,
+ "stations": "Krupkat Landing,Goeppert-Mayer Station"
+ },
+ {
+ "name": "Haritis",
+ "pos_x": -48.46875,
+ "pos_y": 46.28125,
+ "pos_z": -75.6875,
+ "stations": "Tem Dock,Napier Port,Karl Diesel Beacon"
+ },
+ {
+ "name": "Harm",
+ "pos_x": 15.34375,
+ "pos_y": -119.03125,
+ "pos_z": -84.5625,
+ "stations": "Gentil Hub,Rosse's Progress,Finlay-Freundlich Ring,Maskelyne Settlement"
+ },
+ {
+ "name": "Harma",
+ "pos_x": -99.25,
+ "pos_y": -100.96875,
+ "pos_z": 20.40625,
+ "stations": "Gabriel Enterprise,Muhammad Ibn Battuta Point,Celebi City,Maybury City"
+ },
+ {
+ "name": "Harmana",
+ "pos_x": 119.25,
+ "pos_y": 96.34375,
+ "pos_z": 16.28125,
+ "stations": "Fulton Settlement,Lousma Platform,Adams Beacon,Quaglia Barracks"
+ },
+ {
+ "name": "Haroingori",
+ "pos_x": 75.875,
+ "pos_y": -20.78125,
+ "pos_z": 95.34375,
+ "stations": "Gabriel Dock,Crown Ring,Card Terminal,Cummings Holdings,Dall Ring"
+ },
+ {
+ "name": "Harow",
+ "pos_x": 86.21875,
+ "pos_y": 99.65625,
+ "pos_z": -124.46875,
+ "stations": "Crown Depot,Dunbar Settlement"
+ },
+ {
+ "name": "Harowar",
+ "pos_x": 26.53125,
+ "pos_y": -218.5,
+ "pos_z": 27.8125,
+ "stations": "Krigstein Relay,Lyne Relay"
+ },
+ {
+ "name": "Harpai",
+ "pos_x": -56.875,
+ "pos_y": 117.0625,
+ "pos_z": 11.65625,
+ "stations": "Fontenay Landing,Taylor Hub,Carlisle Keep"
+ },
+ {
+ "name": "Harpakhe",
+ "pos_x": 119.84375,
+ "pos_y": 14.1875,
+ "pos_z": -91.40625,
+ "stations": "Garay Works"
+ },
+ {
+ "name": "Harpas",
+ "pos_x": 54.5,
+ "pos_y": 112.65625,
+ "pos_z": -122.28125,
+ "stations": "Zamyatin Enterprise,Bridger Orbital,Gordon Settlement,Maury Bastion,Galois Plant"
+ },
+ {
+ "name": "Harpaso",
+ "pos_x": -11.25,
+ "pos_y": -197.34375,
+ "pos_z": 27.78125,
+ "stations": "Kopal Camp,Bothezat Landing"
+ },
+ {
+ "name": "Harpetet",
+ "pos_x": -38.09375,
+ "pos_y": -61.15625,
+ "pos_z": 140.0625,
+ "stations": "Liouville Asylum,North Penal colony"
+ },
+ {
+ "name": "Harpulidna",
+ "pos_x": 65.09375,
+ "pos_y": -21.65625,
+ "pos_z": 14.78125,
+ "stations": "Lopez-Alegria Enterprise,Tilman Installation,Seddon Ring,Feoktistov Ring"
+ },
+ {
+ "name": "Harpuligni",
+ "pos_x": -53.9375,
+ "pos_y": -41.40625,
+ "pos_z": -96.1875,
+ "stations": "Kier Escape,Kingsbury Mine"
+ },
+ {
+ "name": "Harram",
+ "pos_x": -6.03125,
+ "pos_y": -0.75,
+ "pos_z": 111.625,
+ "stations": "Olivas Mine"
+ },
+ {
+ "name": "Harrigi",
+ "pos_x": -50.71875,
+ "pos_y": -93.71875,
+ "pos_z": -114.375,
+ "stations": "Polya Station,Stuart's Progress,Morris Gateway,Hiroyuki Orbital"
+ },
+ {
+ "name": "Harrigus",
+ "pos_x": 129.6875,
+ "pos_y": -15,
+ "pos_z": 3.40625,
+ "stations": "Stephenson Colony,von Helmont Dock"
+ },
+ {
+ "name": "Hasaka",
+ "pos_x": -151.53125,
+ "pos_y": -85.5625,
+ "pos_z": -44.65625,
+ "stations": "Barjavel Mine,Roberts Platform"
+ },
+ {
+ "name": "Hasattra",
+ "pos_x": -141.5625,
+ "pos_y": -64.75,
+ "pos_z": 35.3125,
+ "stations": "Thirsk Colony"
+ },
+ {
+ "name": "Hastar",
+ "pos_x": -79.5,
+ "pos_y": 80.46875,
+ "pos_z": 57.15625,
+ "stations": "Alvares Enterprise"
+ },
+ {
+ "name": "Haste",
+ "pos_x": 76.65625,
+ "pos_y": 10.34375,
+ "pos_z": -76.8125,
+ "stations": "Roberts Ring,Hermite Co-operative,Marlowe Keep,Merchiston Terminal,Wilson Vision"
+ },
+ {
+ "name": "Hastyat",
+ "pos_x": -78.875,
+ "pos_y": -65.46875,
+ "pos_z": -12.65625,
+ "stations": "Laplace Landing"
+ },
+ {
+ "name": "HAT 94-00572",
+ "pos_x": -27.90625,
+ "pos_y": 15,
+ "pos_z": -77.03125,
+ "stations": "Musa al-Khwarizmi Station,Hooke Station"
+ },
+ {
+ "name": "Hatici",
+ "pos_x": 109.375,
+ "pos_y": -106.5,
+ "pos_z": -80.65625,
+ "stations": "Pogson Landing,Campbell Mines,White Refinery"
+ },
+ {
+ "name": "Hatis",
+ "pos_x": -54.3125,
+ "pos_y": -7.4375,
+ "pos_z": 149.09375,
+ "stations": "Zhen Dock,Baraniecki Prospect"
+ },
+ {
+ "name": "Hatmehi",
+ "pos_x": 49.71875,
+ "pos_y": -156.5625,
+ "pos_z": -66.21875,
+ "stations": "Shaw Ring"
+ },
+ {
+ "name": "Hatmehing",
+ "pos_x": -36.8125,
+ "pos_y": -118.1875,
+ "pos_z": 41.625,
+ "stations": "Gorey Ring,Hevelius Terminal,Stephens Terminal,Joy Gateway,Elbakyan Port,Celsius Dock,Schiaparelli Dock,Uto Terminal,Malocello Depot,Peary Beacon,Grijalva Holdings"
+ },
+ {
+ "name": "Hatmehit",
+ "pos_x": 54,
+ "pos_y": -114.84375,
+ "pos_z": 125.8125,
+ "stations": "Mandel Station,Hopkinson Depot"
+ },
+ {
+ "name": "Hau Ku",
+ "pos_x": 55.875,
+ "pos_y": -130.03125,
+ "pos_z": -47.0625,
+ "stations": "Stone Station,Connes Hub"
+ },
+ {
+ "name": "Hau Yu",
+ "pos_x": -51.03125,
+ "pos_y": -173.0625,
+ "pos_z": 56.71875,
+ "stations": "Barmin's Claim,Gutierrez Works"
+ },
+ {
+ "name": "Haush",
+ "pos_x": -5.3125,
+ "pos_y": -147.5,
+ "pos_z": -33.34375,
+ "stations": "Korlevic Depot,Johnson Settlement,Zacuto Relay"
+ },
+ {
+ "name": "Haushu",
+ "pos_x": 88.4375,
+ "pos_y": -50.40625,
+ "pos_z": -128.0625,
+ "stations": "Nakaya's Claim"
+ },
+ {
+ "name": "Hava",
+ "pos_x": -54.84375,
+ "pos_y": 84.09375,
+ "pos_z": -3.46875,
+ "stations": "Lee Landing,Zebrowski Hub"
+ },
+ {
+ "name": "Havalokul",
+ "pos_x": -129.21875,
+ "pos_y": 16.3125,
+ "pos_z": 56.875,
+ "stations": "Fidalgo Dock,Garnier Survey,Finch Point,Franklin City"
+ },
+ {
+ "name": "Havans",
+ "pos_x": 124.9375,
+ "pos_y": -220.40625,
+ "pos_z": -56.625,
+ "stations": "Mason Enterprise,Gustafsson Orbital,Tan Mines,Legendre Vision,Cooper Point"
+ },
+ {
+ "name": "Havanth",
+ "pos_x": -112.03125,
+ "pos_y": -20.46875,
+ "pos_z": -42.875,
+ "stations": "Rutherford Mine,Hodgkinson Horizons"
+ },
+ {
+ "name": "Havared",
+ "pos_x": 96.84375,
+ "pos_y": -179.875,
+ "pos_z": -0.40625,
+ "stations": "Albumasar Hub,Brashear Terminal,Boyer Hub,Vuia Survey,Boucher Settlement"
+ },
+ {
+ "name": "Havasupai",
+ "pos_x": -88.75,
+ "pos_y": -76.75,
+ "pos_z": -39.625,
+ "stations": "Lovelace Port,Tuan Colony"
+ },
+ {
+ "name": "Havat",
+ "pos_x": -107,
+ "pos_y": 15.3125,
+ "pos_z": -39.71875,
+ "stations": "Gauss Dock,Piaget Landing"
+ },
+ {
+ "name": "Haya Utres",
+ "pos_x": 42.4375,
+ "pos_y": -118.03125,
+ "pos_z": 57.0625,
+ "stations": "Moffat Platform,Kranz Station,Porco Point"
+ },
+ {
+ "name": "Hayanzib",
+ "pos_x": 57.8125,
+ "pos_y": -99.9375,
+ "pos_z": 123.28125,
+ "stations": "Dalgarno City"
+ },
+ {
+ "name": "Hayar",
+ "pos_x": 19.5625,
+ "pos_y": 113.53125,
+ "pos_z": 20.21875,
+ "stations": "Springer Hub,Andersson Ring,Miller Enterprise,Ford City,Pratchett Enterprise,Lerner Gateway,Remec Station,McQuay Holdings,Fraser Installation"
+ },
+ {
+ "name": "Hayikoku",
+ "pos_x": 67.53125,
+ "pos_y": 27.28125,
+ "pos_z": -149.90625,
+ "stations": "Joliot-Curie Retreat,Perry Survey"
+ },
+ {
+ "name": "Hayimshis",
+ "pos_x": 96.5,
+ "pos_y": -81.125,
+ "pos_z": 78.5,
+ "stations": "Giancola Holdings,Wigura Refinery"
+ },
+ {
+ "name": "Hayimstae",
+ "pos_x": 32.875,
+ "pos_y": -38.46875,
+ "pos_z": -44.75,
+ "stations": "Sellings Base,Vaugh Hub,Perga Point"
+ },
+ {
+ "name": "Hazel",
+ "pos_x": -11.71875,
+ "pos_y": -26.46875,
+ "pos_z": -21.84375,
+ "stations": "Krikalev Dock,New Boracay,Stewart Landing"
+ },
+ {
+ "name": "HD 137388",
+ "pos_x": 92.25,
+ "pos_y": -43.59375,
+ "pos_z": 77.15625,
+ "stations": "Delsanti Vision"
+ },
+ {
+ "name": "HD 13931",
+ "pos_x": -90.40625,
+ "pos_y": -39.875,
+ "pos_z": -105.125,
+ "stations": "Hernandez Colony"
+ },
+ {
+ "name": "HD 208496",
+ "pos_x": 66.125,
+ "pos_y": -160,
+ "pos_z": 133.9375,
+ "stations": "Schwarzschild Prospect,De Dock,Grant Vision,McKean Silo"
+ },
+ {
+ "name": "HD 222582",
+ "pos_x": -60.8125,
+ "pos_y": -121.71875,
+ "pos_z": 7.15625,
+ "stations": "McMonagle Ring,Lefschetz Silo"
+ },
+ {
+ "name": "HDS 1065",
+ "pos_x": 40.375,
+ "pos_y": 33.3125,
+ "pos_z": -99.96875,
+ "stations": "Zholobov Ring,Deluc Dock,Aleksandrov Gateway,Reynolds Orbital,Usachov Penal colony,Khayyam Port,Stephenson Dock,Surayev Hub,Furukawa Terminal,Wul Landing"
+ },
+ {
+ "name": "HDS 123",
+ "pos_x": 4.71875,
+ "pos_y": -99.28125,
+ "pos_z": 0,
+ "stations": "Humphreys Base,Foglio Port,Abetti Platform"
+ },
+ {
+ "name": "HDS 1353",
+ "pos_x": -6.28125,
+ "pos_y": 60.3125,
+ "pos_z": -57.90625,
+ "stations": "Quimby Gateway,Landsteiner Port,Garratt Orbital,Lundwall Keep"
+ },
+ {
+ "name": "HDS 1879",
+ "pos_x": -38.125,
+ "pos_y": 69.1875,
+ "pos_z": -17.09375,
+ "stations": "Kronecker Dock,Shaikh Dock"
+ },
+ {
+ "name": "HDS 2154",
+ "pos_x": 25.03125,
+ "pos_y": 55.375,
+ "pos_z": 92.125,
+ "stations": "Garratt Platform,Ellison Reach,Burnet Landing"
+ },
+ {
+ "name": "HDS 3083",
+ "pos_x": -74.25,
+ "pos_y": -25.5625,
+ "pos_z": 13.625,
+ "stations": "Kubasov Landing,Belyayev Hub"
+ },
+ {
+ "name": "HDS 3215",
+ "pos_x": 31.53125,
+ "pos_y": -54.21875,
+ "pos_z": 40.78125,
+ "stations": "Lloyd Wright Orbital,Witt Survey"
+ },
+ {
+ "name": "He Bo",
+ "pos_x": -34.5,
+ "pos_y": 69.28125,
+ "pos_z": -14.71875,
+ "stations": "Roddenberry Station,Gerrold Holdings,Pashin Settlement,Nordenskiold Point,Krylov Ring,Carpini Base,Finch Enterprise,Wylie Hub"
+ },
+ {
+ "name": "He Po",
+ "pos_x": 85.8125,
+ "pos_y": -189.875,
+ "pos_z": -23.21875,
+ "stations": "Barnwell Mines"
+ },
+ {
+ "name": "He Pola",
+ "pos_x": 62.78125,
+ "pos_y": -113.8125,
+ "pos_z": 110.4375,
+ "stations": "Titius Orbital,Pilyugin Orbital,Auzout Orbital,Brashear Relay"
+ },
+ {
+ "name": "He Pora",
+ "pos_x": 73.09375,
+ "pos_y": 53.09375,
+ "pos_z": 89.15625,
+ "stations": "Wul Orbital,Petaja Station,Bisson Enterprise,Westerfeld Arsenal,Irens Enterprise,Kress Station,Kennan Station,Drake Port,Rodrigues Port"
+ },
+ {
+ "name": "He Qinga",
+ "pos_x": 129.34375,
+ "pos_y": -240.34375,
+ "pos_z": -24.125,
+ "stations": "Ghez Landing"
+ },
+ {
+ "name": "He Qingalu",
+ "pos_x": 38.71875,
+ "pos_y": 100.8125,
+ "pos_z": 96.71875,
+ "stations": "Card City"
+ },
+ {
+ "name": "He Qingga",
+ "pos_x": 12.59375,
+ "pos_y": 116.59375,
+ "pos_z": -89.125,
+ "stations": "Weber Platform,Payne Port,Stebler Prospect"
+ },
+ {
+ "name": "He Qingola",
+ "pos_x": 124.53125,
+ "pos_y": -83.9375,
+ "pos_z": -34.3125,
+ "stations": "Hall Hub,Shinjo City"
+ },
+ {
+ "name": "He Qiong",
+ "pos_x": 112.46875,
+ "pos_y": -16.34375,
+ "pos_z": -40.96875,
+ "stations": "Doi Survey,Hornby Port,Wilson Survey"
+ },
+ {
+ "name": "He Xian Di",
+ "pos_x": -62.8125,
+ "pos_y": 25.5625,
+ "pos_z": -162.78125,
+ "stations": "White Landing,Schachner Camp,Borel Installation"
+ },
+ {
+ "name": "He Xian Gu",
+ "pos_x": -52.09375,
+ "pos_y": 3.09375,
+ "pos_z": 122.90625,
+ "stations": "Alvares Beacon,Siegel Dock,Reed Base"
+ },
+ {
+ "name": "He Xians",
+ "pos_x": -52.6875,
+ "pos_y": -90.5,
+ "pos_z": 68.0625,
+ "stations": "Kraft Port,Jones Terminal,Anderson Beacon,Lomonosov's Progress,Cleve Landing,Hirn Terminal"
+ },
+ {
+ "name": "He Xincians",
+ "pos_x": 95,
+ "pos_y": -200.375,
+ "pos_z": 19.15625,
+ "stations": "Oosterhoff's Claim"
+ },
+ {
+ "name": "He Xingo",
+ "pos_x": 104.09375,
+ "pos_y": -100.25,
+ "pos_z": 84.5,
+ "stations": "Tusi City,Dugan Dock,Kent Beacon"
+ },
+ {
+ "name": "Heart Sector IR-V b2-0",
+ "pos_x": -5303.78125,
+ "pos_y": 130.34375,
+ "pos_z": -5305.40625,
+ "stations": "Farsight Expedition Base"
+ },
+ {
+ "name": "Hebe",
+ "pos_x": -47.15625,
+ "pos_y": -37.1875,
+ "pos_z": -55.09375,
+ "stations": "Land Mines,Tanner Landing,Tognini City"
+ },
+ {
+ "name": "Hecate",
+ "pos_x": -56,
+ "pos_y": -25.125,
+ "pos_z": -44.28125,
+ "stations": "RJH1972,William Bertram Ayres"
+ },
+ {
+ "name": "Hedeinichs",
+ "pos_x": 13.6875,
+ "pos_y": -53.5625,
+ "pos_z": -68.375,
+ "stations": "Cook Base,Higginbotham Dock"
+ },
+ {
+ "name": "Hedete",
+ "pos_x": 107.28125,
+ "pos_y": 87.6875,
+ "pos_z": 15.9375,
+ "stations": "Leopold Mines,Heisenberg Colony,Melvill Horizons"
+ },
+ {
+ "name": "Hedetes",
+ "pos_x": -51.625,
+ "pos_y": 139.46875,
+ "pos_z": 8.9375,
+ "stations": "Hennen Port,Olivas Orbital,Oleskiw Settlement,Bresnik Landing,Bering Relay"
+ },
+ {
+ "name": "Hedetet",
+ "pos_x": -30.40625,
+ "pos_y": -59.96875,
+ "pos_z": -54.6875,
+ "stations": "Hermite Dock,du Fresne Gateway,Forfait Port,Nourse Ring,Lewis Hub,Brillant Terminal,Piercy Gateway,Vlamingh Enterprise,Alvarez de Pineda Ring,Hand Legacy,Cardano Enterprise,Rawat Enterprise"
+ },
+ {
+ "name": "Heget",
+ "pos_x": 100.625,
+ "pos_y": -144.46875,
+ "pos_z": 30.75,
+ "stations": "Beekman Orbital,Fodder's Retreat,Zindell Survey,Linge Enterprise"
+ },
+ {
+ "name": "Hehe",
+ "pos_x": 14.75,
+ "pos_y": -18.53125,
+ "pos_z": -101.875,
+ "stations": "Rutherford Hub,Sadi Carnot Ring,Somerset Gateway,Koontz Hub,Plexico Survey"
+ },
+ {
+ "name": "Hehebeche",
+ "pos_x": 100,
+ "pos_y": -16.25,
+ "pos_z": 85.6875,
+ "stations": "Fabricius Vision,Aaronson Landing,Elwood Works"
+ },
+ {
+ "name": "Hehen Bulu",
+ "pos_x": 19.71875,
+ "pos_y": 46.84375,
+ "pos_z": -110.28125,
+ "stations": "Cugnot Station"
+ },
+ {
+ "name": "Hehenes",
+ "pos_x": 97.21875,
+ "pos_y": -7.5,
+ "pos_z": -125.90625,
+ "stations": "Normand Mines,Gottlob Frege Dock"
+ },
+ {
+ "name": "Heheng",
+ "pos_x": 68.21875,
+ "pos_y": 46.4375,
+ "pos_z": 67.6875,
+ "stations": "Onufriyenko Terminal,Clark Enterprise,Disch Prospect,Stefanyshyn-Piper Hub,Minkowski Keep"
+ },
+ {
+ "name": "Heheng De",
+ "pos_x": -119.34375,
+ "pos_y": 31.71875,
+ "pos_z": -9.625,
+ "stations": "Mayer Hub,Oefelein Refinery"
+ },
+ {
+ "name": "Heidjalan",
+ "pos_x": -45.71875,
+ "pos_y": -101.875,
+ "pos_z": 54.46875,
+ "stations": "Gemar Depot,Nikolayev Settlement"
+ },
+ {
+ "name": "Heidruna",
+ "pos_x": 12.625,
+ "pos_y": 112.28125,
+ "pos_z": -21.59375,
+ "stations": "Howard Mines,Illy Landing"
+ },
+ {
+ "name": "Heidubres",
+ "pos_x": -42.75,
+ "pos_y": 95.90625,
+ "pos_z": 39.46875,
+ "stations": "Irens Point"
+ },
+ {
+ "name": "Heidumani",
+ "pos_x": 122.65625,
+ "pos_y": -62.84375,
+ "pos_z": 75.78125,
+ "stations": "Frazetta Point"
+ },
+ {
+ "name": "Heike",
+ "pos_x": -76.96875,
+ "pos_y": 71.90625,
+ "pos_z": 69.1875,
+ "stations": "Brunel City,Braun Enterprise,McMonagle Orbital,Cabana Dock,Lyell Port,Haignere Orbital,Werber Legacy,Velho's Pride"
+ },
+ {
+ "name": "Heikegani",
+ "pos_x": 84.84375,
+ "pos_y": 0.4375,
+ "pos_z": 57.59375,
+ "stations": "Panshin Port,Oswald's Pride,Bartlett Gateway,Lawhead Enterprise,Mitropoulos Hub,Strzelecki Enterprise,Taylor Prospect"
+ },
+ {
+ "name": "Heikegar",
+ "pos_x": 154.53125,
+ "pos_y": -59.78125,
+ "pos_z": -51.15625,
+ "stations": "van de Hulst Port,Kotzebue Vision,Apianus Hangar,Bolger Arsenal"
+ },
+ {
+ "name": "Heikena",
+ "pos_x": 149.53125,
+ "pos_y": -32.40625,
+ "pos_z": 73.78125,
+ "stations": "Garrido Gateway,Hahn Installation,Somerville Base"
+ },
+ {
+ "name": "Heilelang",
+ "pos_x": 166.25,
+ "pos_y": -107.375,
+ "pos_z": -17.40625,
+ "stations": "Suydam Orbital,Aguirre's Inheritance,Offutt Depot,Saha Terminal,Whelan Vision"
+ },
+ {
+ "name": "Heiltsu",
+ "pos_x": -183.21875,
+ "pos_y": 87.65625,
+ "pos_z": 16.71875,
+ "stations": "Zoline Dock,Schouten Port,Dayuan Prospect"
+ },
+ {
+ "name": "Heimanes",
+ "pos_x": 97.78125,
+ "pos_y": -250.375,
+ "pos_z": 23.40625,
+ "stations": "Bingzhen Survey,Dowty Base,Niven Plant"
+ },
+ {
+ "name": "Heimr",
+ "pos_x": -41.9375,
+ "pos_y": 6.6875,
+ "pos_z": 169.21875,
+ "stations": "Clute Landing,Krylov Station"
+ },
+ {
+ "name": "Heimrita",
+ "pos_x": 11.21875,
+ "pos_y": -142.625,
+ "pos_z": 23.9375,
+ "stations": "Corben Station,Mather Hub,Cousteau Works,Sopwith Orbital,Przhevalsky Depot"
+ },
+ {
+ "name": "Heite",
+ "pos_x": 89.09375,
+ "pos_y": 113.875,
+ "pos_z": 45.03125,
+ "stations": "Herzfeld Terminal,Ostwald Outpost"
+ },
+ {
+ "name": "Heitjmaramu",
+ "pos_x": -38.40625,
+ "pos_y": 80.625,
+ "pos_z": 92.625,
+ "stations": "Chu City"
+ },
+ {
+ "name": "Heitsi",
+ "pos_x": -56,
+ "pos_y": -14.96875,
+ "pos_z": 143.90625,
+ "stations": "Rushd Platform,Yegorov Works,Phillifent Point"
+ },
+ {
+ "name": "Hel",
+ "pos_x": -66.03125,
+ "pos_y": -33.84375,
+ "pos_z": -69.96875,
+ "stations": "Jones Orbital,Williams Silo,Samuda Vision"
+ },
+ {
+ "name": "Helgaedi",
+ "pos_x": 82.96875,
+ "pos_y": 26.25,
+ "pos_z": 33.78125,
+ "stations": "Binet Gateway,Belyayev Park,Joliot-Curie Enterprise,Williamson Barracks"
+ },
+ {
+ "name": "Helgarai",
+ "pos_x": 70.15625,
+ "pos_y": -6.125,
+ "pos_z": 117.5625,
+ "stations": "Sharp Reach"
+ },
+ {
+ "name": "Helgardu",
+ "pos_x": 99.40625,
+ "pos_y": -12.46875,
+ "pos_z": 4,
+ "stations": "Wafa Barracks,Slade Terminal,Hogan Ring,Galiano Terminal,Alexandrov Colony"
+ },
+ {
+ "name": "Helgoland",
+ "pos_x": -9510.21875,
+ "pos_y": -908.5,
+ "pos_z": 19783.78125,
+ "stations": "Stoertebeker Dock"
+ },
+ {
+ "name": "Helheim",
+ "pos_x": -90.6875,
+ "pos_y": 60.71875,
+ "pos_z": 132.21875,
+ "stations": "Noether Outpost"
+ },
+ {
+ "name": "Helios",
+ "pos_x": 32.34375,
+ "pos_y": 42.1875,
+ "pos_z": -11.28125,
+ "stations": "Euclid City,McArthur Ring,Hale Depot,Cardano Colony,Aldiss Dock"
+ },
+ {
+ "name": "Hellapa",
+ "pos_x": -84.71875,
+ "pos_y": -74.75,
+ "pos_z": 92.15625,
+ "stations": "Karl Diesel Ring,Onufriyenko Terminal,Hume Arsenal"
+ },
+ {
+ "name": "Helveti",
+ "pos_x": -31.40625,
+ "pos_y": -153.78125,
+ "pos_z": -81.875,
+ "stations": "Macedo Enterprise"
+ },
+ {
+ "name": "Helvetitj",
+ "pos_x": -23.1875,
+ "pos_y": 80.03125,
+ "pos_z": 61.84375,
+ "stations": "Friend Orbital"
+ },
+ {
+ "name": "Helvi",
+ "pos_x": 127.0625,
+ "pos_y": 56.5,
+ "pos_z": 102.625,
+ "stations": "Moresby Terminal"
+ },
+ {
+ "name": "Hemairus",
+ "pos_x": -64.375,
+ "pos_y": 106.03125,
+ "pos_z": 26.125,
+ "stations": "Shiner Enterprise,Vernadsky Port"
+ },
+ {
+ "name": "Hemait",
+ "pos_x": 164.21875,
+ "pos_y": -196.28125,
+ "pos_z": 49.53125,
+ "stations": "Flamsteed Base,Burnham Settlement,Griffiths Hub"
+ },
+ {
+ "name": "Hemak",
+ "pos_x": -33.25,
+ "pos_y": -88.78125,
+ "pos_z": 49.5,
+ "stations": "Ross Colony,Serebrov Port,Roberts Keep"
+ },
+ {
+ "name": "Hemaki",
+ "pos_x": -38.875,
+ "pos_y": -22.5625,
+ "pos_z": -115.5,
+ "stations": "Kizim Station,Battuta Gateway,Laval Terminal,Wegener Station"
+ },
+ {
+ "name": "Hemang",
+ "pos_x": -39,
+ "pos_y": 73.09375,
+ "pos_z": -113.875,
+ "stations": "Williams City,Ziemianski Dock,Baffin Silo,Collins Installation"
+ },
+ {
+ "name": "Hemangu",
+ "pos_x": 131.09375,
+ "pos_y": -38.21875,
+ "pos_z": -92.78125,
+ "stations": "Nikolayev Ring"
+ },
+ {
+ "name": "Hemaskas",
+ "pos_x": 33.5,
+ "pos_y": -139.625,
+ "pos_z": 10.15625,
+ "stations": "Ghez Prospect,Brouwer Refinery,Osterbrock Platform,Baillaud Landing"
+ },
+ {
+ "name": "Hembal",
+ "pos_x": -140.5625,
+ "pos_y": -69.375,
+ "pos_z": 117.09375,
+ "stations": "Ray Point,Danvers Reach"
+ },
+ {
+ "name": "Hembe",
+ "pos_x": 147.59375,
+ "pos_y": 74.78125,
+ "pos_z": 8,
+ "stations": "McBride Hub,Savitskaya City,Treshchov Dock,MacCurdy Base,Kingsbury's Folly"
+ },
+ {
+ "name": "Hementrim",
+ "pos_x": -160.4375,
+ "pos_y": 13.90625,
+ "pos_z": -70.125,
+ "stations": "Godel Station"
+ },
+ {
+ "name": "Hemez",
+ "pos_x": -13.875,
+ "pos_y": -176.8125,
+ "pos_z": 20.15625,
+ "stations": "Abe Holdings,Vavrova Mines,Oikawa Horizons"
+ },
+ {
+ "name": "Henes",
+ "pos_x": -48.0625,
+ "pos_y": 163.5625,
+ "pos_z": 8.78125,
+ "stations": "Lorrah's Claim,Tavares Settlement,MacLeod Silo"
+ },
+ {
+ "name": "Henet",
+ "pos_x": 148.53125,
+ "pos_y": -180.53125,
+ "pos_z": 74.125,
+ "stations": "Reinmuth Port,Sheepshanks Terminal,Israel Dock,Skjellerup Settlement"
+ },
+ {
+ "name": "Heng",
+ "pos_x": -39.59375,
+ "pos_y": -124,
+ "pos_z": -101.15625,
+ "stations": "Dirichlet Enterprise,Ricci Orbital,Eisenstein City,Varthema Prospect,Barnes Landing"
+ },
+ {
+ "name": "Hengubu",
+ "pos_x": -5.8125,
+ "pos_y": -119.9375,
+ "pos_z": -92.3125,
+ "stations": "Wandrei's Claim"
+ },
+ {
+ "name": "Hengyang",
+ "pos_x": 99.1875,
+ "pos_y": -1.03125,
+ "pos_z": 120.03125,
+ "stations": "Ibold Survey,Meyrink Legacy,Lenthall Enterprise"
+ },
+ {
+ "name": "Hepa",
+ "pos_x": -29.4375,
+ "pos_y": 34.46875,
+ "pos_z": 21.53125,
+ "stations": "Miller Refinery,Watt-Evans Depot,Hendel Refinery"
+ },
+ {
+ "name": "Hephaestus",
+ "pos_x": -9521.40625,
+ "pos_y": -907.3125,
+ "pos_z": 19801.65625,
+ "stations": "Malik Station,Millennium Point"
+ },
+ {
+ "name": "Heptis",
+ "pos_x": 1.59375,
+ "pos_y": -143.21875,
+ "pos_z": 127.78125,
+ "stations": "Hirayama Base,Merrill Terminal,Tank Port"
+ },
+ {
+ "name": "Hera",
+ "pos_x": -36.8125,
+ "pos_y": 55.59375,
+ "pos_z": -17.96875,
+ "stations": "Sellings Beacon,Bounds Station,Goulart Dock,MacLeod Port,Greenleaf Base"
+ },
+ {
+ "name": "Herce",
+ "pos_x": 4.1875,
+ "pos_y": -139.625,
+ "pos_z": 128.875,
+ "stations": "Myasishchev Landing,Jean Survey,Mechain Colony,Rolland Beacon"
+ },
+ {
+ "name": "Herci",
+ "pos_x": 68.84375,
+ "pos_y": -43.34375,
+ "pos_z": -0.8125,
+ "stations": "Junlong Beacon,Anvil Mines,Elcano Platform"
+ },
+ {
+ "name": "Herculis Sector IR-W c1-7",
+ "pos_x": -56.53125,
+ "pos_y": 83.40625,
+ "pos_z": 79,
+ "stations": "de Balboa Vision,Chorel Survey"
+ },
+ {
+ "name": "Herculis Sector NN-T b3-3",
+ "pos_x": -53.71875,
+ "pos_y": 84.59375,
+ "pos_z": 69.21875,
+ "stations": "O'Donnell's Inheritance,Ziemkiewicz's Claim,Hamilton Works"
+ },
+ {
+ "name": "Herculis Sector YJ-A c16",
+ "pos_x": -59.90625,
+ "pos_y": 96.3125,
+ "pos_z": 7.625,
+ "stations": "Detention Ship Gamma"
+ },
+ {
+ "name": "Hercuna",
+ "pos_x": 159.78125,
+ "pos_y": -195.5625,
+ "pos_z": 55.53125,
+ "stations": "Matthaus Olbers Relay"
+ },
+ {
+ "name": "Hercuni",
+ "pos_x": 71.40625,
+ "pos_y": -116.21875,
+ "pos_z": 78.90625,
+ "stations": "Mayor Terminal"
+ },
+ {
+ "name": "Herebitinta",
+ "pos_x": 23.3125,
+ "pos_y": 58,
+ "pos_z": 149.34375,
+ "stations": "Thompson Enterprise,Padalka Orbital,Hadfield Landing,Al-Farabi Landing"
+ },
+ {
+ "name": "Hereda",
+ "pos_x": 78.125,
+ "pos_y": 80.09375,
+ "pos_z": 18.75,
+ "stations": "Fischer Platform,Watson Platform"
+ },
+ {
+ "name": "Herengul",
+ "pos_x": 58.125,
+ "pos_y": -121.96875,
+ "pos_z": 37.1875,
+ "stations": "Shapley Horizons,Romer Station"
+ },
+ {
+ "name": "Herete",
+ "pos_x": -95.625,
+ "pos_y": 117.125,
+ "pos_z": -84.40625,
+ "stations": "Dayuan Freeport,Hui Survey,Nansen Mines"
+ },
+ {
+ "name": "Heretia",
+ "pos_x": 58.96875,
+ "pos_y": -62.375,
+ "pos_z": -92.96875,
+ "stations": "Schiltberger Reformatory,Meaney Platform"
+ },
+ {
+ "name": "Herfang",
+ "pos_x": 92.65625,
+ "pos_y": -4.5,
+ "pos_z": -31.09375,
+ "stations": "Zalyotin Landing,Clervoy Port"
+ },
+ {
+ "name": "Herfjongo",
+ "pos_x": 88.3125,
+ "pos_y": 46.3125,
+ "pos_z": -20.4375,
+ "stations": "Walker Prospect,Nourse Vision,Phillpotts Orbital,Anderson Estate"
+ },
+ {
+ "name": "Herfjoturr",
+ "pos_x": -69.375,
+ "pos_y": -147.25,
+ "pos_z": -46.4375,
+ "stations": "Landsteiner Orbital,Appel Landing,Linge Installation"
+ },
+ {
+ "name": "Heria Pared",
+ "pos_x": -169.90625,
+ "pos_y": -52.0625,
+ "pos_z": 73.34375,
+ "stations": "Wilder Platform,Drummond Penal colony"
+ },
+ {
+ "name": "Herishep",
+ "pos_x": -11.25,
+ "pos_y": -19.09375,
+ "pos_z": -66.125,
+ "stations": "Harris Platform"
+ },
+ {
+ "name": "Herlio",
+ "pos_x": -94.71875,
+ "pos_y": -6.5,
+ "pos_z": -1.34375,
+ "stations": "Ostwald Outpost,Johnson Colony,Petaja's Folly"
+ },
+ {
+ "name": "Herme",
+ "pos_x": -36,
+ "pos_y": -159.78125,
+ "pos_z": 38.40625,
+ "stations": "Suydam Orbital,Gill Dock,Nearchus Horizons,Dingle Port,Herodotus Point"
+ },
+ {
+ "name": "Hermid",
+ "pos_x": -92,
+ "pos_y": 114.5,
+ "pos_z": 17.0625,
+ "stations": "DeLucas Survey,Pythagoras Landing,Diesel Settlement"
+ },
+ {
+ "name": "Hermoduroua",
+ "pos_x": 97.4375,
+ "pos_y": -1,
+ "pos_z": -59.78125,
+ "stations": "Blaha Vision,Snyder Vision,Bluford Depot,Low Horizons,Melvill Hub"
+ },
+ {
+ "name": "Hermoricaur",
+ "pos_x": 133.15625,
+ "pos_y": -207.0625,
+ "pos_z": 107.15625,
+ "stations": "Lyot Stop"
+ },
+ {
+ "name": "Hermoth",
+ "pos_x": 95.1875,
+ "pos_y": -33.21875,
+ "pos_z": 49.90625,
+ "stations": "Schouten Base,Cousin Outpost"
+ },
+ {
+ "name": "HerniMara",
+ "pos_x": -71,
+ "pos_y": -21.9375,
+ "pos_z": 100.03125,
+ "stations": "Lagrange Market,Kozlov Orbital,Fox City,He Port,Eudoxus Orbital,Garfinkle Landing,Almagro Base,Baxter Arsenal"
+ },
+ {
+ "name": "Hernir",
+ "pos_x": -38.34375,
+ "pos_y": -96.59375,
+ "pos_z": -69.71875,
+ "stations": "Weyl Beacon,Gunn Ring,Serling Beacon,Rawat Hub,Hahn Settlement"
+ },
+ {
+ "name": "Hernkondha",
+ "pos_x": 127.96875,
+ "pos_y": -49.6875,
+ "pos_z": 57.6875,
+ "stations": "Malchiodi Settlement,Isherwood Landing"
+ },
+ {
+ "name": "Hernkopa",
+ "pos_x": 58.40625,
+ "pos_y": -221.90625,
+ "pos_z": 95.96875,
+ "stations": "Mobius Station,Penrose Settlement,Vakhmistrov Landing,Wilhelm von Struve Base"
+ },
+ {
+ "name": "Hernobo",
+ "pos_x": 182.53125,
+ "pos_y": -127.875,
+ "pos_z": -12.65625,
+ "stations": "Kubokawa Port,Ahnert-Rohlfs Ring,Lewis Ring,Thomas' Folly"
+ },
+ {
+ "name": "Hernovacle",
+ "pos_x": 119.875,
+ "pos_y": -153,
+ "pos_z": 72.65625,
+ "stations": "Abel Dock"
+ },
+ {
+ "name": "Herthans",
+ "pos_x": 42.71875,
+ "pos_y": -121.5625,
+ "pos_z": 41.84375,
+ "stations": "Lavochkin Station,Henry Vision,Wegener Terminal"
+ },
+ {
+ "name": "Herthe",
+ "pos_x": 57.4375,
+ "pos_y": -12.71875,
+ "pos_z": 2.15625,
+ "stations": "Brackett Installation,Noguchi Dock,Walheim Enterprise,Bernoulli Hub"
+ },
+ {
+ "name": "Herti",
+ "pos_x": 25.78125,
+ "pos_y": -210,
+ "pos_z": 63.5,
+ "stations": "Pinto's Folly,Ahnert-Rohlfs Port,Dilworth Landing"
+ },
+ {
+ "name": "Heru",
+ "pos_x": -151.90625,
+ "pos_y": -44.5625,
+ "pos_z": 14.75,
+ "stations": "Caselberg's Claim"
+ },
+ {
+ "name": "Herus",
+ "pos_x": 38.96875,
+ "pos_y": 60.4375,
+ "pos_z": -123.375,
+ "stations": "Grigson Port"
+ },
+ {
+ "name": "Heryshaf",
+ "pos_x": 144.03125,
+ "pos_y": -106.59375,
+ "pos_z": 23.4375,
+ "stations": "Sheremetevsky Dock,Neugebauer Prospect,Chawla Mines,Dugan Installation,Silverberg's Progress"
+ },
+ {
+ "name": "HERZ 10688",
+ "pos_x": -4.3125,
+ "pos_y": -74.3125,
+ "pos_z": -42.8125,
+ "stations": "Elvstrom Silo,URZ 1871,Krylov Base"
+ },
+ {
+ "name": "HERZ 13422",
+ "pos_x": 74.03125,
+ "pos_y": 55.625,
+ "pos_z": -29.0625,
+ "stations": "Hassanein Hub,Darboux Barracks,Clark Dock,Collins Settlement"
+ },
+ {
+ "name": "HERZ 2910",
+ "pos_x": -63.78125,
+ "pos_y": -92.90625,
+ "pos_z": 37.4375,
+ "stations": "Garrett Settlement"
+ },
+ {
+ "name": "HERZ 589",
+ "pos_x": 32.21875,
+ "pos_y": -61.625,
+ "pos_z": -75.0625,
+ "stations": "Barnes Dock"
+ },
+ {
+ "name": "Hesa",
+ "pos_x": 89.1875,
+ "pos_y": 46.59375,
+ "pos_z": -15.8125,
+ "stations": "Lyell Dock,Bova Settlement,Al-Kashi Installation"
+ },
+ {
+ "name": "Hesanthen",
+ "pos_x": -14.28125,
+ "pos_y": 103.21875,
+ "pos_z": -76.84375,
+ "stations": "Volk Enterprise,Goulart's Claim,Lasswitz Sanctuary,Tucker Silo"
+ },
+ {
+ "name": "Hesates",
+ "pos_x": 4.9375,
+ "pos_y": 43.71875,
+ "pos_z": 89.875,
+ "stations": "Collins Prospect,Bartlett Landing,Herbert Landing,Vancouver Base"
+ },
+ {
+ "name": "Hesatsu",
+ "pos_x": -27.53125,
+ "pos_y": -56.75,
+ "pos_z": 73.125,
+ "stations": "Morris Prospect,MacVicar Orbital"
+ },
+ {
+ "name": "Hesqui Xing",
+ "pos_x": -148.59375,
+ "pos_y": 1.5625,
+ "pos_z": 40.125,
+ "stations": "Agnesi Colony,Nelson Penitentiary"
+ },
+ {
+ "name": "Hesquix",
+ "pos_x": -57.71875,
+ "pos_y": -179.5,
+ "pos_z": -1.84375,
+ "stations": "Tarter Terminal"
+ },
+ {
+ "name": "Het",
+ "pos_x": -128.46875,
+ "pos_y": 33.9375,
+ "pos_z": -19.46875,
+ "stations": "Schweickart Terminal,Fujimori Hub,Taylor Point"
+ },
+ {
+ "name": "Hetmehi",
+ "pos_x": 69.34375,
+ "pos_y": 118.09375,
+ "pos_z": 64.375,
+ "stations": "Ricci Prospect,Paulo da Gama Port"
+ },
+ {
+ "name": "Hevelisci",
+ "pos_x": -83.4375,
+ "pos_y": 123.03125,
+ "pos_z": 33.90625,
+ "stations": "Savorgnan de Brazza Platform,Hume Terminal"
+ },
+ {
+ "name": "Heverduduna",
+ "pos_x": 60.34375,
+ "pos_y": -79.4375,
+ "pos_z": -71.96875,
+ "stations": "Jacquard Orbital,Lu Gateway,Engle Platform,Archer Silo,Rasmussen Beacon"
+ },
+ {
+ "name": "Heveri",
+ "pos_x": 69.96875,
+ "pos_y": 25.96875,
+ "pos_z": 17.09375,
+ "stations": "Birdseye Enterprise,Gulyaev Holdings,Wiberg Hub,Merbold Depot,Davy Terminal"
+ },
+ {
+ "name": "Hevernaku",
+ "pos_x": -101.84375,
+ "pos_y": -115.3125,
+ "pos_z": -28.6875,
+ "stations": "Witt Dock"
+ },
+ {
+ "name": "Heverty",
+ "pos_x": 111.6875,
+ "pos_y": -61.875,
+ "pos_z": 55.15625,
+ "stations": "Woodroffe Gateway,Cavendish Base,Emshwiller's Inheritance"
+ },
+ {
+ "name": "Hez Pef",
+ "pos_x": 111.15625,
+ "pos_y": 0.8125,
+ "pos_z": 62.78125,
+ "stations": "Thirsk Orbital,Fuca Installation"
+ },
+ {
+ "name": "Hez Pei",
+ "pos_x": -0.75,
+ "pos_y": 110.75,
+ "pos_z": -40.5,
+ "stations": "Rothfuss Landing"
+ },
+ {
+ "name": "Hez Ur",
+ "pos_x": 149.96875,
+ "pos_y": 23.65625,
+ "pos_z": -85.03125,
+ "stations": "Jeschke Orbital,Valdes Horizons"
+ },
+ {
+ "name": "HG 7-206",
+ "pos_x": 7.90625,
+ "pos_y": -35.8125,
+ "pos_z": -68.46875,
+ "stations": "Davidson Landing"
+ },
+ {
+ "name": "Hida",
+ "pos_x": -24.9375,
+ "pos_y": -159.84375,
+ "pos_z": -62.625,
+ "stations": "Glushko's Folly,Dishoeck Mines,Pilcher Platform"
+ },
+ {
+ "name": "Hidar",
+ "pos_x": 26.15625,
+ "pos_y": -55.03125,
+ "pos_z": -141.875,
+ "stations": "Gerrold Terminal,Yourkevitch Penal colony"
+ },
+ {
+ "name": "Hidatsa",
+ "pos_x": -5.625,
+ "pos_y": 13.96875,
+ "pos_z": -111.0625,
+ "stations": "Laue Terminal,Fuglesang Terminal,Ciferri Enterprise"
+ },
+ {
+ "name": "Hikenk",
+ "pos_x": 97.375,
+ "pos_y": -10.3125,
+ "pos_z": 100.5625,
+ "stations": "Giles Orbital,Hell Hub"
+ },
+ {
+ "name": "Hill Pa Hsi",
+ "pos_x": 29.46875,
+ "pos_y": -1.6875,
+ "pos_z": 25.375,
+ "stations": "Reilly Terminal,Wisoff Terminal,Curie Gateway,Malerba Gateway,McArthur Beacon,Teng-hui Gateway,Nicolet Point,Mohri Depot"
+ },
+ {
+ "name": "Hilla",
+ "pos_x": 108.21875,
+ "pos_y": 45.1875,
+ "pos_z": 48.71875,
+ "stations": "Siemens Vision,Durrance Hub,Flint Point,Temple Hub,Salam Point"
+ },
+ {
+ "name": "Hillaunges",
+ "pos_x": 206.375,
+ "pos_y": -131.90625,
+ "pos_z": 83.21875,
+ "stations": "Argelander Port,Powers Vision,Wrangel Installation,Kuo Horizons"
+ },
+ {
+ "name": "Hima",
+ "pos_x": -15.1875,
+ "pos_y": 20.625,
+ "pos_z": -114.46875,
+ "stations": "Meucci Port,White Enterprise"
+ },
+ {
+ "name": "Himanes",
+ "pos_x": -79.0625,
+ "pos_y": 3.4375,
+ "pos_z": 109.84375,
+ "stations": "Ochoa Port,Stefanyshyn-Piper Port,Redi Laboratory,Saavedra Penal colony"
+ },
+ {
+ "name": "Himanezgana",
+ "pos_x": 16.28125,
+ "pos_y": -31.53125,
+ "pos_z": -137.625,
+ "stations": "Filipchenko Terminal"
+ },
+ {
+ "name": "Himang",
+ "pos_x": -90.03125,
+ "pos_y": -4.78125,
+ "pos_z": 89.625,
+ "stations": "Kapp Landing,Slayton Hub,Armstrong Colony,Humboldt Port"
+ },
+ {
+ "name": "Himaphan",
+ "pos_x": -71.53125,
+ "pos_y": -14.4375,
+ "pos_z": 108.78125,
+ "stations": "Quaglia Estate"
+ },
+ {
+ "name": "Himardisc",
+ "pos_x": 83.25,
+ "pos_y": -47.84375,
+ "pos_z": 114.3125,
+ "stations": "Barmin's Inheritance,Carrington Base"
+ },
+ {
+ "name": "Himashi",
+ "pos_x": 115.125,
+ "pos_y": -98.59375,
+ "pos_z": -80.65625,
+ "stations": "Mies van der Rohe Plant,Carrington Estate,Kaiser Installation,Piano Base,Ricci Camp"
+ },
+ {
+ "name": "Himavat",
+ "pos_x": 23.4375,
+ "pos_y": 51.90625,
+ "pos_z": 133.90625,
+ "stations": "Akers Landing,Knight Holdings,Hodgkinson Prospect"
+ },
+ {
+ "name": "Himavisci",
+ "pos_x": 51.125,
+ "pos_y": 53.34375,
+ "pos_z": -121.03125,
+ "stations": "Silves Landing,von Bellingshausen Lab,Lambert Arsenal,Johnson Installation,Ashton Settlement"
+ },
+ {
+ "name": "Himbalbaye",
+ "pos_x": -20.3125,
+ "pos_y": 87.21875,
+ "pos_z": 70.59375,
+ "stations": "Hugh Estate,Teng Refinery,Coney Installation"
+ },
+ {
+ "name": "Himbawegas",
+ "pos_x": 35.03125,
+ "pos_y": 116.125,
+ "pos_z": 51.6875,
+ "stations": "Kaku Relay,Ewald Base"
+ },
+ {
+ "name": "Hinepon",
+ "pos_x": 138.9375,
+ "pos_y": -182.46875,
+ "pos_z": -52.09375,
+ "stations": "Fabricius Point,Ilyushin's Claim"
+ },
+ {
+ "name": "Hinook",
+ "pos_x": -85.5,
+ "pos_y": 15.5,
+ "pos_z": 103.625,
+ "stations": "Ricci Estate,Fidalgo Prospect"
+ },
+ {
+ "name": "Hinus",
+ "pos_x": 89.4375,
+ "pos_y": -23.15625,
+ "pos_z": -92.09375,
+ "stations": "Cowper Stop"
+ },
+ {
+ "name": "HIP 100035",
+ "pos_x": 35.84375,
+ "pos_y": -110.21875,
+ "pos_z": 155.15625,
+ "stations": "Carroll Dock,Cherry Hub"
+ },
+ {
+ "name": "HIP 100063",
+ "pos_x": 107.59375,
+ "pos_y": -130.65625,
+ "pos_z": 165.71875,
+ "stations": "Fukushima Hub,Gurshtein Orbital,Riebe Bastion"
+ },
+ {
+ "name": "HIP 100072",
+ "pos_x": -75.9375,
+ "pos_y": -48.34375,
+ "pos_z": 92.9375,
+ "stations": "al-Khowarizmi Hub,Besonders Holdings,Bounds Station,Escobar Survey,Oliver Orbital"
+ },
+ {
+ "name": "HIP 10012",
+ "pos_x": 34.5625,
+ "pos_y": -176.9375,
+ "pos_z": -44.75,
+ "stations": "Hooker Gateway"
+ },
+ {
+ "name": "HIP 100179",
+ "pos_x": 31.53125,
+ "pos_y": -86.5625,
+ "pos_z": 119.875,
+ "stations": "Thierree Dock,Weaver Station,Almagro Survey"
+ },
+ {
+ "name": "HIP 100225",
+ "pos_x": 75.03125,
+ "pos_y": -89.71875,
+ "pos_z": 112.71875,
+ "stations": "Niemeyer Terminal,Bretnor Arsenal,Block Vision"
+ },
+ {
+ "name": "HIP 100233",
+ "pos_x": -35.6875,
+ "pos_y": -66.40625,
+ "pos_z": 107.4375,
+ "stations": "Daimler Market,Birdseye Silo,Bethke Vision,Berners-Lee Vision"
+ },
+ {
+ "name": "HIP 100235",
+ "pos_x": -96.875,
+ "pos_y": -73.625,
+ "pos_z": 134.15625,
+ "stations": "Clayton Mines,North's Claim"
+ },
+ {
+ "name": "HIP 100280",
+ "pos_x": 70.0625,
+ "pos_y": -66.6875,
+ "pos_z": 79.8125,
+ "stations": "Narlikar Hub,Nijland Landing,Takahashi Bastion"
+ },
+ {
+ "name": "HIP 100284",
+ "pos_x": -130.0625,
+ "pos_y": 67.78125,
+ "pos_z": -66,
+ "stations": "Sutcliffe City,Eskridge Dock,H. G. Wells Settlement,Rayhan al-Biruni Terminal,Bellamy Point"
+ },
+ {
+ "name": "HIP 1003",
+ "pos_x": -3.03125,
+ "pos_y": -155.5625,
+ "pos_z": 23.53125,
+ "stations": "Opik Vision"
+ },
+ {
+ "name": "HIP 100341",
+ "pos_x": -153.1875,
+ "pos_y": 11.8125,
+ "pos_z": 21.78125,
+ "stations": "Wells Vision,Lupoff Enterprise,McKay Dock,Ayerdhal Beacon"
+ },
+ {
+ "name": "HIP 100359",
+ "pos_x": 144.6875,
+ "pos_y": -114.15625,
+ "pos_z": 130.03125,
+ "stations": "Lewis Vision"
+ },
+ {
+ "name": "HIP 100379",
+ "pos_x": 62.0625,
+ "pos_y": -81.59375,
+ "pos_z": 103.3125,
+ "stations": "Cesar Janssen Gateway,Graham Ring,Naddoddur Relay"
+ },
+ {
+ "name": "HIP 100405",
+ "pos_x": 34.53125,
+ "pos_y": -85.8125,
+ "pos_z": 116.28125,
+ "stations": "McKie Park,Baily Terminal,d'Allonville Station,Cooper's Folly"
+ },
+ {
+ "name": "HIP 100449",
+ "pos_x": -16,
+ "pos_y": -92.125,
+ "pos_z": 137.5625,
+ "stations": "Fleming Enterprise,Potrykus Dock,Hannu Beacon,Overmyer Hub"
+ },
+ {
+ "name": "HIP 100466",
+ "pos_x": 116.3125,
+ "pos_y": -129.21875,
+ "pos_z": 158.5625,
+ "stations": "Theodorsen Depot,Danjon Port"
+ },
+ {
+ "name": "HIP 10050",
+ "pos_x": -47.5,
+ "pos_y": -80.84375,
+ "pos_z": -78.78125,
+ "stations": "Martins Terminal,Amnuel Terminal,Thurston Keep,Williams Terminal,Abnett Hub,Grigson Keep,Chern Enterprise,Littlewood City,Toll Dock,Grimwood Orbital,Norton Terminal,Zebrowski Terminal,Dunyach Depot,Moran Forum"
+ },
+ {
+ "name": "HIP 100500",
+ "pos_x": -139.21875,
+ "pos_y": -28.25,
+ "pos_z": 74.75,
+ "stations": "Swigert Terminal,Wolfe's Folly,Chomsky Dock,Prunariu Landing"
+ },
+ {
+ "name": "HIP 100539",
+ "pos_x": 155.65625,
+ "pos_y": -149.03125,
+ "pos_z": 177.1875,
+ "stations": "Rho Platform"
+ },
+ {
+ "name": "HIP 100685",
+ "pos_x": -64.90625,
+ "pos_y": -87.9375,
+ "pos_z": 140.28125,
+ "stations": "Durrance City,Ross Dock,Lloyd Oasis"
+ },
+ {
+ "name": "HIP 10079",
+ "pos_x": -103.75,
+ "pos_y": -48.71875,
+ "pos_z": -116.625,
+ "stations": "Kirtley Dock,Bulychev Colony,Gardner Enterprise"
+ },
+ {
+ "name": "HIP 100792",
+ "pos_x": -147.46875,
+ "pos_y": -54.65625,
+ "pos_z": 109.90625,
+ "stations": "Taylor Observatory"
+ },
+ {
+ "name": "HIP 100818",
+ "pos_x": 9.6875,
+ "pos_y": -96.46875,
+ "pos_z": 133.59375,
+ "stations": "Gotz's Claim"
+ },
+ {
+ "name": "HIP 100891",
+ "pos_x": 129.9375,
+ "pos_y": -112.9375,
+ "pos_z": 129.96875,
+ "stations": "Fukushima Dock,Ban Terminal"
+ },
+ {
+ "name": "HIP 100895",
+ "pos_x": -96.0625,
+ "pos_y": -59.15625,
+ "pos_z": 103.34375,
+ "stations": "Burke Port,Hyecho Port,Ellison Enterprise,Shiner Depot,Olsen Arsenal"
+ },
+ {
+ "name": "HIP 100934",
+ "pos_x": 50.21875,
+ "pos_y": -106.21875,
+ "pos_z": 137.40625,
+ "stations": "Jenkins Station,Naylor Prospect,Kovalevskaya Keep"
+ },
+ {
+ "name": "HIP 10096",
+ "pos_x": 82.5,
+ "pos_y": -216.1875,
+ "pos_z": -18.71875,
+ "stations": "Brothers Terminal,Jones Colony,Chaffee Platform,Goryu Beacon,Gurshtein Horizons"
+ },
+ {
+ "name": "HIP 100967",
+ "pos_x": 41.4375,
+ "pos_y": -141.90625,
+ "pos_z": 188.59375,
+ "stations": "Kraft Depot,Blaauw Base,Serling Depot,Sucharitkul Reach"
+ },
+ {
+ "name": "HIP 100968",
+ "pos_x": 171.6875,
+ "pos_y": -114.375,
+ "pos_z": 122.96875,
+ "stations": "Foster Relay,Vlaicu Settlement"
+ },
+ {
+ "name": "HIP 100970",
+ "pos_x": -104.9375,
+ "pos_y": -24.96875,
+ "pos_z": 56.6875,
+ "stations": "Lambert Arsenal,Melnick Hub"
+ },
+ {
+ "name": "HIP 101031",
+ "pos_x": 71.8125,
+ "pos_y": -117.15625,
+ "pos_z": 147.3125,
+ "stations": "Oosterhoff Station,Havilland Survey,Oikawa Penal colony"
+ },
+ {
+ "name": "HIP 101136",
+ "pos_x": -107.65625,
+ "pos_y": -74.4375,
+ "pos_z": 123.71875,
+ "stations": "Warner's Progress"
+ },
+ {
+ "name": "HIP 101153",
+ "pos_x": 36.15625,
+ "pos_y": -121.375,
+ "pos_z": 159.25,
+ "stations": "Pond Prospect,Pilyugin Port,Trujillo's Folly"
+ },
+ {
+ "name": "HIP 10123",
+ "pos_x": 59.71875,
+ "pos_y": -183.3125,
+ "pos_z": -25.6875,
+ "stations": "Burnham Market,Marcy Barracks,Rich Gateway,Lemaitre's Claim,Riebe Reach,Velho Base,Poncelet Observatory"
+ },
+ {
+ "name": "HIP 101268",
+ "pos_x": -144.28125,
+ "pos_y": -8.6875,
+ "pos_z": 39.21875,
+ "stations": "Ramaswamy Mines"
+ },
+ {
+ "name": "HIP 101274",
+ "pos_x": -13.40625,
+ "pos_y": -75.5,
+ "pos_z": 105.03125,
+ "stations": "Leeuwenhoek Landing"
+ },
+ {
+ "name": "HIP 1013",
+ "pos_x": -18.5625,
+ "pos_y": -228.21875,
+ "pos_z": 26.6875,
+ "stations": "Lindstrand Terminal,Altuna Mines"
+ },
+ {
+ "name": "HIP 101304",
+ "pos_x": 111.6875,
+ "pos_y": -157.59375,
+ "pos_z": 192.5,
+ "stations": "Hoerner's Folly"
+ },
+ {
+ "name": "HIP 10137",
+ "pos_x": 191.28125,
+ "pos_y": -178.96875,
+ "pos_z": 97.40625,
+ "stations": "Bickel Vision"
+ },
+ {
+ "name": "HIP 101432",
+ "pos_x": 0.03125,
+ "pos_y": -77.75,
+ "pos_z": 104.3125,
+ "stations": "Bolton Port,Mooz Orbital,von Biela Market,Mayer Dock"
+ },
+ {
+ "name": "HIP 101461",
+ "pos_x": 12.15625,
+ "pos_y": -90.375,
+ "pos_z": 118.78125,
+ "stations": "Qushji Plant"
+ },
+ {
+ "name": "HIP 101549",
+ "pos_x": -22.03125,
+ "pos_y": -97.75,
+ "pos_z": 133.71875,
+ "stations": "Swigert Hub,Kaku Colony,Midgeley Park"
+ },
+ {
+ "name": "HIP 101587",
+ "pos_x": 22.125,
+ "pos_y": -94.6875,
+ "pos_z": 121.65625,
+ "stations": "Hynek Terminal,Nomen Landing,Barbaro Installation,Hulse Ring,la Cosa Silo"
+ },
+ {
+ "name": "HIP 101597",
+ "pos_x": -107.1875,
+ "pos_y": -40.4375,
+ "pos_z": 71.5625,
+ "stations": "Murray Colony,Garn Dock,Garan Hub,Borel's Progress,Binnie Terminal,Malerba Hub,Still Hub,Tani Enterprise,Lucretius City,Wohler Barracks,Gelfand Horizons,Hoffman Orbital,Thorne Terminal,Weston Vista"
+ },
+ {
+ "name": "HIP 101613",
+ "pos_x": -195.3125,
+ "pos_y": 52.5,
+ "pos_z": -36.6875,
+ "stations": "Harness Stop,Lorrah Reach"
+ },
+ {
+ "name": "HIP 101620",
+ "pos_x": -121.75,
+ "pos_y": -64.46875,
+ "pos_z": 105.46875,
+ "stations": "Resnick Terminal,Fowler Legacy"
+ },
+ {
+ "name": "HIP 101628",
+ "pos_x": 151.59375,
+ "pos_y": -119,
+ "pos_z": 131.78125,
+ "stations": "Friedrich Peters Point,Darwin Stop"
+ },
+ {
+ "name": "HIP 101636",
+ "pos_x": 51.6875,
+ "pos_y": -136.125,
+ "pos_z": 170.84375,
+ "stations": "Loewy Survey,Brooks Penal colony"
+ },
+ {
+ "name": "HIP 101726",
+ "pos_x": 6.71875,
+ "pos_y": -74.84375,
+ "pos_z": 96.78125,
+ "stations": "Mason Dock,Ivens Gateway,Biesbroeck Station,Bickel Terminal,Shoemaker City,Bigourdan Gateway,Crown Enterprise,Belyanin Relay,Cousteau Barracks,Stefansson's Inheritance,Stevens Horizons"
+ },
+ {
+ "name": "HIP 10175",
+ "pos_x": -45.78125,
+ "pos_y": -93.5,
+ "pos_z": -83.90625,
+ "stations": "Stefanyshyn-Piper Station,Lucretius Gateway,Davis Arsenal,Fu Observatory,Donaldson Prospect"
+ },
+ {
+ "name": "HIP 101760",
+ "pos_x": -80.8125,
+ "pos_y": -80.34375,
+ "pos_z": 117.59375,
+ "stations": "Haldeman II Enterprise,Wallin Dock,MacCurdy Penal colony,Nesvadba's Inheritance"
+ },
+ {
+ "name": "HIP 101785",
+ "pos_x": -55.28125,
+ "pos_y": -93.1875,
+ "pos_z": 130.03125,
+ "stations": "Ferguson Horizons"
+ },
+ {
+ "name": "HIP 101806",
+ "pos_x": 52.9375,
+ "pos_y": -97.84375,
+ "pos_z": 119.125,
+ "stations": "Peebles Dock"
+ },
+ {
+ "name": "HIP 101812",
+ "pos_x": 67.6875,
+ "pos_y": -146.90625,
+ "pos_z": 180.65625,
+ "stations": "Otomo Port,Sitter Relay"
+ },
+ {
+ "name": "HIP 101846",
+ "pos_x": 23.3125,
+ "pos_y": -76.96875,
+ "pos_z": 96.28125,
+ "stations": "Biesbroeck Dock,Frazetta Ring,Fowler Ring,Edmondson Terminal,Hartmann Station,Betancourt Base,Blaschke Enterprise,Peters Legacy"
+ },
+ {
+ "name": "HIP 101852",
+ "pos_x": -84.96875,
+ "pos_y": -62.65625,
+ "pos_z": 94.21875,
+ "stations": "Kelly Colony"
+ },
+ {
+ "name": "HIP 101854",
+ "pos_x": 126.625,
+ "pos_y": -139.46875,
+ "pos_z": 161.625,
+ "stations": "Albumasar Outpost"
+ },
+ {
+ "name": "HIP 101856",
+ "pos_x": -90.21875,
+ "pos_y": -42.625,
+ "pos_z": 68.96875,
+ "stations": "Vasilyev Landing"
+ },
+ {
+ "name": "HIP 101857",
+ "pos_x": 86.5,
+ "pos_y": -120.46875,
+ "pos_z": 143.03125,
+ "stations": "Leberecht Tempel Vision,Brooks Hub,Brosnatch Installation"
+ },
+ {
+ "name": "HIP 101893",
+ "pos_x": -162.53125,
+ "pos_y": -27.96875,
+ "pos_z": 60.4375,
+ "stations": "Harding's Progress"
+ },
+ {
+ "name": "HIP 101904",
+ "pos_x": -40.96875,
+ "pos_y": -104.53125,
+ "pos_z": 141.0625,
+ "stations": "Carstensz Vision,Akers Depot"
+ },
+ {
+ "name": "HIP 101933",
+ "pos_x": -104.875,
+ "pos_y": -40.59375,
+ "pos_z": 67.71875,
+ "stations": "Sternberg Landing,Roberts' Claim,Foden Refinery"
+ },
+ {
+ "name": "HIP 102016",
+ "pos_x": -171.625,
+ "pos_y": -6.125,
+ "pos_z": 32.125,
+ "stations": "Makarov Gateway,Smith Enterprise,Everest Landing,Teller Beacon"
+ },
+ {
+ "name": "HIP 102018",
+ "pos_x": -39.3125,
+ "pos_y": -74.25,
+ "pos_z": 100.5625,
+ "stations": "Whitelaw Point,Reiter Enterprise,Frimout City"
+ },
+ {
+ "name": "HIP 102081",
+ "pos_x": -171.28125,
+ "pos_y": -23.46875,
+ "pos_z": 53.25,
+ "stations": "Fibonacci Port"
+ },
+ {
+ "name": "HIP 102128",
+ "pos_x": 87.5,
+ "pos_y": -87.3125,
+ "pos_z": 98.90625,
+ "stations": "Dishoeck Arsenal,Stein Mines,Yerka Reach"
+ },
+ {
+ "name": "HIP 102259",
+ "pos_x": 130.34375,
+ "pos_y": -124.96875,
+ "pos_z": 140.375,
+ "stations": "Mooz Orbital,Neville's Folly,Young City,Laurent Hub"
+ },
+ {
+ "name": "HIP 102406",
+ "pos_x": 97.96875,
+ "pos_y": -126.78125,
+ "pos_z": 145.6875,
+ "stations": "Gora Prospect,Harvey-Smith Landing,Brera Settlement"
+ },
+ {
+ "name": "HIP 10245",
+ "pos_x": -108.21875,
+ "pos_y": -41,
+ "pos_z": -118.90625,
+ "stations": "Malchiodi Enterprise,Tanaka Enterprise"
+ },
+ {
+ "name": "HIP 102548",
+ "pos_x": 60.5,
+ "pos_y": -133.53125,
+ "pos_z": 157.0625,
+ "stations": "Becvar's Pride,Weill Sanctuary"
+ },
+ {
+ "name": "HIP 1026",
+ "pos_x": -152.90625,
+ "pos_y": -49.15625,
+ "pos_z": -76.1875,
+ "stations": "Shinn Prospect,Citi Installation"
+ },
+ {
+ "name": "HIP 102655",
+ "pos_x": -102.28125,
+ "pos_y": -85.5,
+ "pos_z": 114.21875,
+ "stations": "Gutierrez Installation"
+ },
+ {
+ "name": "HIP 102664",
+ "pos_x": -60.59375,
+ "pos_y": -65.8125,
+ "pos_z": 85.96875,
+ "stations": "Finney Beacon"
+ },
+ {
+ "name": "HIP 102725",
+ "pos_x": 115.40625,
+ "pos_y": -150,
+ "pos_z": 170,
+ "stations": "Kirby Outpost,Davis Enterprise"
+ },
+ {
+ "name": "HIP 102807",
+ "pos_x": -136.78125,
+ "pos_y": -53.375,
+ "pos_z": 77.0625,
+ "stations": "Dorsett Colony,Lousma Works,Pavlov Dock,Andree Settlement"
+ },
+ {
+ "name": "HIP 102815",
+ "pos_x": -154.15625,
+ "pos_y": -33.78125,
+ "pos_z": 54.96875,
+ "stations": "Artin Landing,Rae's Inheritance,Jordan Prospect"
+ },
+ {
+ "name": "HIP 102817",
+ "pos_x": -33.125,
+ "pos_y": -88.25,
+ "pos_z": 109.125,
+ "stations": "Stairs Port,Riemann Station,Cyrrhus Enterprise,Sewell Settlement"
+ },
+ {
+ "name": "HIP 102865",
+ "pos_x": 36.53125,
+ "pos_y": -132,
+ "pos_z": 154.625,
+ "stations": "Ziljak Prospect,Bradfield Platform,Abel Beacon"
+ },
+ {
+ "name": "HIP 102868",
+ "pos_x": 149.21875,
+ "pos_y": -118.875,
+ "pos_z": 128.78125,
+ "stations": "Corben Relay"
+ },
+ {
+ "name": "HIP 102869",
+ "pos_x": -49.40625,
+ "pos_y": -103.03125,
+ "pos_z": 127.625,
+ "stations": "Farouk Dock"
+ },
+ {
+ "name": "HIP 102895",
+ "pos_x": 32.59375,
+ "pos_y": -82.6875,
+ "pos_z": 95.84375,
+ "stations": "Barmin Terminal"
+ },
+ {
+ "name": "HIP 102923",
+ "pos_x": -117.59375,
+ "pos_y": -61.25,
+ "pos_z": 83.15625,
+ "stations": "Shepard Dock,Legendre Horizons,Mille Settlement,Cardano Works"
+ },
+ {
+ "name": "HIP 102924",
+ "pos_x": 143.71875,
+ "pos_y": -131.9375,
+ "pos_z": 144.625,
+ "stations": "Gonnessiat Depot,Condit Stop,Ross' Claim"
+ },
+ {
+ "name": "HIP 102962",
+ "pos_x": 87.65625,
+ "pos_y": -153.75,
+ "pos_z": 175.125,
+ "stations": "Siddha Prospect,Youll Platform"
+ },
+ {
+ "name": "HIP 103031",
+ "pos_x": 85.625,
+ "pos_y": -118,
+ "pos_z": 132.40625,
+ "stations": "Riess Station,Schoening Hub,Whymper Prospect"
+ },
+ {
+ "name": "HIP 103048",
+ "pos_x": 45.34375,
+ "pos_y": -146.46875,
+ "pos_z": 169.0625,
+ "stations": "Sagan Vision,Fairey Depot"
+ },
+ {
+ "name": "HIP 103107",
+ "pos_x": 30.8125,
+ "pos_y": -151.5,
+ "pos_z": 175.375,
+ "stations": "Bharadwaj Dock,Common Colony,Chacornac Escape"
+ },
+ {
+ "name": "HIP 103138",
+ "pos_x": -50.53125,
+ "pos_y": -75.5,
+ "pos_z": 92.0625,
+ "stations": "Strekalov Dock,Payette Vision,Curie Beacon,Bradbury Barracks"
+ },
+ {
+ "name": "HIP 103208",
+ "pos_x": 28.53125,
+ "pos_y": -136.59375,
+ "pos_z": 156.96875,
+ "stations": "Louis de Lacaille Orbital,Trujillo Station,Lee Arsenal,Seitter Hub,Baldwin Beacon,Gaspar de Lemos Keep"
+ },
+ {
+ "name": "HIP 10322",
+ "pos_x": -95.65625,
+ "pos_y": -8.3125,
+ "pos_z": -93.03125,
+ "stations": "Linteris Ring,Lousma Observatory,Zewail Bastion"
+ },
+ {
+ "name": "HIP 10325",
+ "pos_x": 89.875,
+ "pos_y": -237.5625,
+ "pos_z": -25.1875,
+ "stations": "Whipple Ring,Skiff Dock,Villarceau Enterprise"
+ },
+ {
+ "name": "HIP 103250",
+ "pos_x": -95,
+ "pos_y": -86.65625,
+ "pos_z": 107,
+ "stations": "Abel Prospect,Teng Colony,Nicolet Survey"
+ },
+ {
+ "name": "HIP 103260",
+ "pos_x": 26.90625,
+ "pos_y": -97.75,
+ "pos_z": 111.53125,
+ "stations": "Stebbins Hub,Paczynski Landing,Fuller Orbital,Regiomontanus' Progress"
+ },
+ {
+ "name": "HIP 103311",
+ "pos_x": -62.21875,
+ "pos_y": -86.59375,
+ "pos_z": 104,
+ "stations": "Wagner Port,Levi-Civita Ring,Weinbaum Station,Clapperton Dock,Godwin Dock"
+ },
+ {
+ "name": "HIP 103361",
+ "pos_x": 51.8125,
+ "pos_y": -109.90625,
+ "pos_z": 123.1875,
+ "stations": "Porco Port,Hertzsprung Mines,Arnold Schwassmann Dock"
+ },
+ {
+ "name": "HIP 103390",
+ "pos_x": 8.46875,
+ "pos_y": -105.375,
+ "pos_z": 120.375,
+ "stations": "Sweet Dock,Ferguson Dock,von Zach Station,Scheerbart Landing,Palmer Relay,Fearn Installation"
+ },
+ {
+ "name": "HIP 103430",
+ "pos_x": 57.21875,
+ "pos_y": -130.25,
+ "pos_z": 145.65625,
+ "stations": "Poyser Dock,Kidinnu Dock,al-Haytham Observatory"
+ },
+ {
+ "name": "HIP 103438",
+ "pos_x": 52,
+ "pos_y": -111.875,
+ "pos_z": 124.875,
+ "stations": "Greenland Base,Moffat Dock,Lewis Prospect"
+ },
+ {
+ "name": "HIP 10346",
+ "pos_x": 120.75,
+ "pos_y": -176.5,
+ "pos_z": 31.5,
+ "stations": "Youll Platform,Battani Station,Aaronson Landing"
+ },
+ {
+ "name": "HIP 103498",
+ "pos_x": 19.0625,
+ "pos_y": -113.46875,
+ "pos_z": 128,
+ "stations": "Heiles Station,Calatrava Vision"
+ },
+ {
+ "name": "HIP 103502",
+ "pos_x": -141.71875,
+ "pos_y": -9.375,
+ "pos_z": 18.1875,
+ "stations": "Francisco de Eliza Terminal,Morris Dock,Lefschetz Beacon,Fancher Hub,Maine Bastion"
+ },
+ {
+ "name": "HIP 103578",
+ "pos_x": 23.1875,
+ "pos_y": -140.84375,
+ "pos_z": 157.96875,
+ "stations": "Consolmagno Mines"
+ },
+ {
+ "name": "HIP 103583",
+ "pos_x": 14.8125,
+ "pos_y": -91.90625,
+ "pos_z": 103.03125,
+ "stations": "Inoda Mines"
+ },
+ {
+ "name": "HIP 103620",
+ "pos_x": 23.75,
+ "pos_y": -87.21875,
+ "pos_z": 97.0625,
+ "stations": "Anderson Terminal"
+ },
+ {
+ "name": "HIP 10363",
+ "pos_x": 122.0625,
+ "pos_y": -106.3125,
+ "pos_z": 64.96875,
+ "stations": "Wegner Installation,Nijland Mines"
+ },
+ {
+ "name": "HIP 10365",
+ "pos_x": -8.03125,
+ "pos_y": -150.28125,
+ "pos_z": -77.09375,
+ "stations": "Coye Dock,August von Steinheil Station,Hawke Bastion"
+ },
+ {
+ "name": "HIP 103654",
+ "pos_x": 60.0625,
+ "pos_y": -77.375,
+ "pos_z": 84.1875,
+ "stations": "Ferguson Penal colony,Pearse Refinery"
+ },
+ {
+ "name": "HIP 103678",
+ "pos_x": -113.5,
+ "pos_y": -42.65625,
+ "pos_z": 52.59375,
+ "stations": "Garan Orbital,Stewart Refinery,Caselberg Hub,Vespucci Beacon"
+ },
+ {
+ "name": "HIP 103710",
+ "pos_x": 0.8125,
+ "pos_y": -108.625,
+ "pos_z": 121.28125,
+ "stations": "Kalam Mine"
+ },
+ {
+ "name": "HIP 103787",
+ "pos_x": -41.875,
+ "pos_y": -79.375,
+ "pos_z": 89.59375,
+ "stations": "Froude Arsenal,Hackworth Terminal,Sommerfeld Base"
+ },
+ {
+ "name": "HIP 103812",
+ "pos_x": -159.0625,
+ "pos_y": -54.5,
+ "pos_z": 65.9375,
+ "stations": "Midgeley Enterprise"
+ },
+ {
+ "name": "HIP 103824",
+ "pos_x": -142.34375,
+ "pos_y": -15.25,
+ "pos_z": 21.71875,
+ "stations": "Bradbury Platform,Shaara Depot"
+ },
+ {
+ "name": "HIP 103854",
+ "pos_x": 87.6875,
+ "pos_y": -163.03125,
+ "pos_z": 177.125,
+ "stations": "Vess Port,Eggen Platform"
+ },
+ {
+ "name": "HIP 103867",
+ "pos_x": 52.75,
+ "pos_y": -86.71875,
+ "pos_z": 93.9375,
+ "stations": "Hoffmeister Terminal,Raleigh Reformatory"
+ },
+ {
+ "name": "HIP 103895",
+ "pos_x": -165.25,
+ "pos_y": -3.90625,
+ "pos_z": 9.4375,
+ "stations": "Galilei Dock"
+ },
+ {
+ "name": "HIP 103896",
+ "pos_x": -58.34375,
+ "pos_y": -93.625,
+ "pos_z": 104.875,
+ "stations": "Anthony Terminal,Monge Landing"
+ },
+ {
+ "name": "HIP 103913",
+ "pos_x": -124.65625,
+ "pos_y": -71.15625,
+ "pos_z": 82.03125,
+ "stations": "Klimuk City,Langford Landing"
+ },
+ {
+ "name": "HIP 103931",
+ "pos_x": -99.90625,
+ "pos_y": -77.90625,
+ "pos_z": 88.46875,
+ "stations": "Moresby Platform,Gromov Colony,Crichton's Inheritance"
+ },
+ {
+ "name": "HIP 104045",
+ "pos_x": -102.75,
+ "pos_y": -90.875,
+ "pos_z": 101.40625,
+ "stations": "Reed Installation,Paez Terminal"
+ },
+ {
+ "name": "HIP 104065",
+ "pos_x": 111.53125,
+ "pos_y": -167.875,
+ "pos_z": 180,
+ "stations": "Wilbert's Wadi"
+ },
+ {
+ "name": "HIP 104071",
+ "pos_x": -28.96875,
+ "pos_y": -122.90625,
+ "pos_z": 134.21875,
+ "stations": "Gerrold Orbital,Soper Landing,Bentham Arsenal"
+ },
+ {
+ "name": "HIP 104124",
+ "pos_x": -138.15625,
+ "pos_y": -35.5625,
+ "pos_z": 41.1875,
+ "stations": "Bertin Dock,H. G. Wells Survey,Anvil Settlement,Steakley Penal colony,Kronecker Relay"
+ },
+ {
+ "name": "HIP 104128",
+ "pos_x": -136.1875,
+ "pos_y": -58.28125,
+ "pos_z": 65.65625,
+ "stations": "Redi Hub"
+ },
+ {
+ "name": "HIP 10413",
+ "pos_x": 94.125,
+ "pos_y": -232.125,
+ "pos_z": -20.09375,
+ "stations": "Naburimannu Colony,Curtis Station,Biermann Reformatory,Rucker Relay"
+ },
+ {
+ "name": "HIP 104163",
+ "pos_x": 27.4375,
+ "pos_y": -160.34375,
+ "pos_z": 172.40625,
+ "stations": "Baillaud Works"
+ },
+ {
+ "name": "HIP 104164",
+ "pos_x": -142.28125,
+ "pos_y": -81.78125,
+ "pos_z": 90.59375,
+ "stations": "Grimwood's Inheritance"
+ },
+ {
+ "name": "HIP 104206",
+ "pos_x": 161,
+ "pos_y": -137.5,
+ "pos_z": 145.375,
+ "stations": "Hansen Dock,Geller Dock,Takamizawa Orbital,Wafer Settlement,Griffiths Survey"
+ },
+ {
+ "name": "HIP 104256",
+ "pos_x": 45.875,
+ "pos_y": -115.90625,
+ "pos_z": 123.59375,
+ "stations": "William Sargent Orbital,Weaver City,Mobius Dock"
+ },
+ {
+ "name": "HIP 104292",
+ "pos_x": 15.21875,
+ "pos_y": -153,
+ "pos_z": 163.3125,
+ "stations": "Lomonosov City,Beatty Enterprise,Homer Gateway,Campbell Arsenal,Behnisch Dock"
+ },
+ {
+ "name": "HIP 104294",
+ "pos_x": -91.40625,
+ "pos_y": -84.53125,
+ "pos_z": 91.28125,
+ "stations": "von Helmont Landing,Moseley Camp"
+ },
+ {
+ "name": "HIP 104318",
+ "pos_x": -94.3125,
+ "pos_y": -55,
+ "pos_z": 59.59375,
+ "stations": "Raleigh Vision"
+ },
+ {
+ "name": "HIP 104331",
+ "pos_x": -72.6875,
+ "pos_y": -77.09375,
+ "pos_z": 82.84375,
+ "stations": "Sacco City,Wheeler Vision"
+ },
+ {
+ "name": "HIP 104349",
+ "pos_x": 146.625,
+ "pos_y": -148.9375,
+ "pos_z": 157.21875,
+ "stations": "Alphonsi Enterprise,Zhu Orbital,Esclangon Station,Ogden's Folly"
+ },
+ {
+ "name": "HIP 104419",
+ "pos_x": -138.03125,
+ "pos_y": -42.65625,
+ "pos_z": 45.8125,
+ "stations": "Baker Relay,Doyle Settlement,Riemann's Progress"
+ },
+ {
+ "name": "HIP 104453",
+ "pos_x": 123.5625,
+ "pos_y": -111.375,
+ "pos_z": 117.15625,
+ "stations": "Jeury Beacon,Huggins Platform,Wilson Orbital"
+ },
+ {
+ "name": "HIP 104471",
+ "pos_x": -126,
+ "pos_y": -47.75,
+ "pos_z": 50.625,
+ "stations": "Carlisle Enterprise,White Station,Mieville Orbital,Cochrane Port,Hartog Enterprise,Ford Holdings,Allen Station"
+ },
+ {
+ "name": "HIP 104476",
+ "pos_x": -51.1875,
+ "pos_y": -111.875,
+ "pos_z": 117.9375,
+ "stations": "Pribylov Dock,Hodgkinson Vision,Stuart Terminal"
+ },
+ {
+ "name": "HIP 10449",
+ "pos_x": -29.78125,
+ "pos_y": -171.84375,
+ "pos_z": -108.8125,
+ "stations": "Bloch's Claim,Sargent's Inheritance"
+ },
+ {
+ "name": "HIP 104504",
+ "pos_x": -47.6875,
+ "pos_y": -107.09375,
+ "pos_z": 112.65625,
+ "stations": "Baillaud Ring,Stein City,Shajn Orbital,Bates' Folly,Krylov Barracks,Rutan's Inheritance"
+ },
+ {
+ "name": "HIP 104549",
+ "pos_x": -54.5625,
+ "pos_y": -112.15625,
+ "pos_z": 117.4375,
+ "stations": "Zettel Survey,Monge Station,Williamson Barracks,Wollheim Camp"
+ },
+ {
+ "name": "HIP 104560",
+ "pos_x": -107.5,
+ "pos_y": -109.21875,
+ "pos_z": 114.125,
+ "stations": "Macdonald Dock,Morgan Landing,Eschbach Hub"
+ },
+ {
+ "name": "HIP 104759",
+ "pos_x": -120.0625,
+ "pos_y": -63.625,
+ "pos_z": 63.9375,
+ "stations": "Coulomb Ring"
+ },
+ {
+ "name": "HIP 104768",
+ "pos_x": 33.1875,
+ "pos_y": -166.1875,
+ "pos_z": 171.4375,
+ "stations": "Kushida Vision,Nouvel Installation"
+ },
+ {
+ "name": "HIP 10483",
+ "pos_x": 66.8125,
+ "pos_y": -210.625,
+ "pos_z": -36.875,
+ "stations": "Borrego Station,Whitney Landing,Naubakht Port"
+ },
+ {
+ "name": "HIP 104856",
+ "pos_x": 100.90625,
+ "pos_y": -111.28125,
+ "pos_z": 115.53125,
+ "stations": "Laming Vision,Sutter Orbital,Karachkina Camp"
+ },
+ {
+ "name": "HIP 104864",
+ "pos_x": -53.9375,
+ "pos_y": -110.09375,
+ "pos_z": 111.53125,
+ "stations": "Hughes Holdings,Laming Colony"
+ },
+ {
+ "name": "HIP 10492",
+ "pos_x": 21.375,
+ "pos_y": -135.46875,
+ "pos_z": -44.125,
+ "stations": "Tarter Dock,Porubcan Dock,Somerville Port,Maitz Port,Molyneux Port,Lomonosov Vision,Watanabe Station"
+ },
+ {
+ "name": "HIP 105062",
+ "pos_x": 9.78125,
+ "pos_y": -93.90625,
+ "pos_z": 94.71875,
+ "stations": "Kulin Vision,Hoerner Vision,Csoma Depot,Karachkina Enterprise"
+ },
+ {
+ "name": "HIP 10510",
+ "pos_x": -62.875,
+ "pos_y": -67.96875,
+ "pos_z": -91.65625,
+ "stations": "Grant Orbital"
+ },
+ {
+ "name": "HIP 105156",
+ "pos_x": -31.375,
+ "pos_y": -101.875,
+ "pos_z": 100.71875,
+ "stations": "Pilcher Mines,Oterma Colony,Greenland Plant,Nilson Legacy"
+ },
+ {
+ "name": "HIP 105192",
+ "pos_x": -153.5,
+ "pos_y": -65.8125,
+ "pos_z": 60.3125,
+ "stations": "Muhammad Ibn Battuta Arsenal,Foden Vista"
+ },
+ {
+ "name": "HIP 105200",
+ "pos_x": -124.84375,
+ "pos_y": -67.78125,
+ "pos_z": 63.21875,
+ "stations": "Weinbaum Enterprise,Holberg Orbital"
+ },
+ {
+ "name": "HIP 105202",
+ "pos_x": -105.6875,
+ "pos_y": -62.875,
+ "pos_z": 58.9375,
+ "stations": "Caidin Hub,Brust Hub,Wells Dock,Turzillo Station,Dummer Orbital,Marques Enterprise,Smith Dock,Makarov Hub,Fearn Barracks,Stillman Station,Nikitin Installation,Kotzebue Holdings"
+ },
+ {
+ "name": "HIP 105261",
+ "pos_x": -87.03125,
+ "pos_y": -72,
+ "pos_z": 68.09375,
+ "stations": "Miletus Freeport,Maler Mine,Farmer Prospect"
+ },
+ {
+ "name": "HIP 10532",
+ "pos_x": -5.875,
+ "pos_y": -133.5,
+ "pos_z": -69.28125,
+ "stations": "Herjulfsson Vision,Huberath Estate"
+ },
+ {
+ "name": "HIP 105359",
+ "pos_x": -94.75,
+ "pos_y": -83.125,
+ "pos_z": 77.8125,
+ "stations": "Lloyd Landing"
+ },
+ {
+ "name": "HIP 105368",
+ "pos_x": -45.875,
+ "pos_y": -100.25,
+ "pos_z": 96.59375,
+ "stations": "Zenbei City,Newcomb Dock,Pryor Plant,Herschel Silo,Lozino-Lozinskiy Station"
+ },
+ {
+ "name": "HIP 105388",
+ "pos_x": 27.15625,
+ "pos_y": -97.59375,
+ "pos_z": 96.875,
+ "stations": "Rigaux Terminal,Samos Hub,Heinkel Port"
+ },
+ {
+ "name": "HIP 105391",
+ "pos_x": 11.53125,
+ "pos_y": -118.625,
+ "pos_z": 116.84375,
+ "stations": "Rogers Hub,Mille Vista,Dashiell Bastion"
+ },
+ {
+ "name": "HIP 105404",
+ "pos_x": 27.125,
+ "pos_y": -102.78125,
+ "pos_z": 101.875,
+ "stations": "Michael Ring,Janjetov Dock"
+ },
+ {
+ "name": "HIP 105408",
+ "pos_x": 18.15625,
+ "pos_y": -147.71875,
+ "pos_z": 145.5,
+ "stations": "Binney Horizons"
+ },
+ {
+ "name": "HIP 105431",
+ "pos_x": -123.34375,
+ "pos_y": -71.28125,
+ "pos_z": 64.125,
+ "stations": "Priestley Station,Beadle Point,Fremont Gateway,Buffett Terminal,Buckey City"
+ },
+ {
+ "name": "HIP 105439",
+ "pos_x": 25.96875,
+ "pos_y": -105.90625,
+ "pos_z": 104.65625,
+ "stations": "Perrine Vision,Wallis Hub,Lagrange Base"
+ },
+ {
+ "name": "HIP 105483",
+ "pos_x": -77.71875,
+ "pos_y": -73.96875,
+ "pos_z": 68.375,
+ "stations": "Kirtley Dock,Nagata Co-operative,Makarov Lab"
+ },
+ {
+ "name": "HIP 1055",
+ "pos_x": -94.0625,
+ "pos_y": -89.40625,
+ "pos_z": -37.5625,
+ "stations": "Moran Stop,Lewis Enterprise"
+ },
+ {
+ "name": "HIP 105510",
+ "pos_x": -153.875,
+ "pos_y": -50.9375,
+ "pos_z": 42.0625,
+ "stations": "Love Settlement,Midgeley Base,Bohnhoff Enterprise"
+ },
+ {
+ "name": "HIP 105511",
+ "pos_x": 151.1875,
+ "pos_y": -119.5,
+ "pos_z": 123.5,
+ "stations": "Naubakht Orbital"
+ },
+ {
+ "name": "HIP 105547",
+ "pos_x": 47.03125,
+ "pos_y": -134.90625,
+ "pos_z": 133.15625,
+ "stations": "Bingzhen Settlement"
+ },
+ {
+ "name": "HIP 105557",
+ "pos_x": -113.53125,
+ "pos_y": 41.21875,
+ "pos_z": -45.6875,
+ "stations": "Perry Base,Mitropoulos' Inheritance,Popov Penal colony"
+ },
+ {
+ "name": "HIP 10556",
+ "pos_x": -102.9375,
+ "pos_y": -37.28125,
+ "pos_z": -115.15625,
+ "stations": "Kuttner Retreat"
+ },
+ {
+ "name": "HIP 105577",
+ "pos_x": 40.125,
+ "pos_y": -160.96875,
+ "pos_z": 157.6875,
+ "stations": "Whitcomb Camp,Sikorsky Port,Metcalf Enterprise"
+ },
+ {
+ "name": "HIP 105606",
+ "pos_x": -0.59375,
+ "pos_y": -87.65625,
+ "pos_z": 84.53125,
+ "stations": "Comino Gateway,Berezovoy Point,Plexico Terminal"
+ },
+ {
+ "name": "HIP 105629",
+ "pos_x": 42.375,
+ "pos_y": -124.0625,
+ "pos_z": 121.75,
+ "stations": "Miller Beacon,al-Din al-Urdi Base"
+ },
+ {
+ "name": "HIP 105639",
+ "pos_x": -127.34375,
+ "pos_y": -100.4375,
+ "pos_z": 89.65625,
+ "stations": "Peary Orbital,Williams Terminal,Mille Arsenal"
+ },
+ {
+ "name": "HIP 10570",
+ "pos_x": 139.78125,
+ "pos_y": -242.03125,
+ "pos_z": 16.09375,
+ "stations": "Cartier Platform,Boyer Prospect,Fernandez's Claim"
+ },
+ {
+ "name": "HIP 105740",
+ "pos_x": -51.90625,
+ "pos_y": -113.875,
+ "pos_z": 105.59375,
+ "stations": "Moore Landing,Kimberlin Orbital"
+ },
+ {
+ "name": "HIP 10579",
+ "pos_x": 22.875,
+ "pos_y": -176.71875,
+ "pos_z": -63.28125,
+ "stations": "Kandel Silo,Sitterly Landing"
+ },
+ {
+ "name": "HIP 105821",
+ "pos_x": -27.9375,
+ "pos_y": -109,
+ "pos_z": 101.65625,
+ "stations": "Lewis Settlement,Weiss Bastion"
+ },
+ {
+ "name": "HIP 10584",
+ "pos_x": -4.1875,
+ "pos_y": -139.8125,
+ "pos_z": -71.25,
+ "stations": "Whitford Colony,Crown Camp,Shute Colony"
+ },
+ {
+ "name": "HIP 105863",
+ "pos_x": 7.0625,
+ "pos_y": -121.8125,
+ "pos_z": 115.65625,
+ "stations": "Shapiro Landing,Kreutz Survey"
+ },
+ {
+ "name": "HIP 10587",
+ "pos_x": 135.5625,
+ "pos_y": -154.6875,
+ "pos_z": 53.875,
+ "stations": "Russell Enterprise,Krylov Stop,Hay Port"
+ },
+ {
+ "name": "HIP 10589",
+ "pos_x": 69.84375,
+ "pos_y": -168.09375,
+ "pos_z": -14.84375,
+ "stations": "Robert Port,Gotz Vision,Herndon Prospect"
+ },
+ {
+ "name": "HIP 105906",
+ "pos_x": -114.40625,
+ "pos_y": 51.40625,
+ "pos_z": -56.125,
+ "stations": "Burkin Silo,Al Saud Dock"
+ },
+ {
+ "name": "HIP 105929",
+ "pos_x": 23.6875,
+ "pos_y": -172.25,
+ "pos_z": 163.65625,
+ "stations": "Campbell Settlement,Sikorsky Base"
+ },
+ {
+ "name": "HIP 106006",
+ "pos_x": -52.9375,
+ "pos_y": -107.90625,
+ "pos_z": 97.09375,
+ "stations": "Quaglia Hangar"
+ },
+ {
+ "name": "HIP 106072",
+ "pos_x": -125.3125,
+ "pos_y": -55.09375,
+ "pos_z": 41.8125,
+ "stations": "Smith Port,Reamy Barracks"
+ },
+ {
+ "name": "HIP 106100",
+ "pos_x": 31.09375,
+ "pos_y": -167.15625,
+ "pos_z": 157.3125,
+ "stations": "Gill Enterprise,Griffiths Holdings,de Kamp Prospect,Miller Prospect"
+ },
+ {
+ "name": "HIP 10611",
+ "pos_x": 91.96875,
+ "pos_y": -170.25,
+ "pos_z": 4.875,
+ "stations": "Plaskett Works,Coye Legacy"
+ },
+ {
+ "name": "HIP 106204",
+ "pos_x": -43.96875,
+ "pos_y": -103.96875,
+ "pos_z": 92.0625,
+ "stations": "Pannekoek Vision"
+ },
+ {
+ "name": "HIP 106208",
+ "pos_x": -32.125,
+ "pos_y": -125.34375,
+ "pos_z": 112.65625,
+ "stations": "Samos Base,Patry Colony"
+ },
+ {
+ "name": "HIP 106213",
+ "pos_x": 42.34375,
+ "pos_y": -82.28125,
+ "pos_z": 79.0625,
+ "stations": "Tanaka Terminal,Shinn City,Forward Hub,Fontenay Dock,Perga Point,Extra Vision,Polya Works,Barnwell Hub,Scalzi Depot"
+ },
+ {
+ "name": "HIP 106288",
+ "pos_x": 15.5625,
+ "pos_y": -158.0625,
+ "pos_z": 145.78125,
+ "stations": "Young Mines"
+ },
+ {
+ "name": "HIP 106296",
+ "pos_x": -134.90625,
+ "pos_y": -54.375,
+ "pos_z": 38.25,
+ "stations": "Pausch Enterprise,Norton Depot,Boas Horizons,Duckworth Ring,Payette Port"
+ },
+ {
+ "name": "HIP 106311",
+ "pos_x": 143.3125,
+ "pos_y": -154.09375,
+ "pos_z": 152.84375,
+ "stations": "Robert Legacy,Homer Mines"
+ },
+ {
+ "name": "HIP 106335",
+ "pos_x": -107.4375,
+ "pos_y": -93.125,
+ "pos_z": 75.53125,
+ "stations": "H. G. Wells Point,MacCurdy Survey,Bolger Survey"
+ },
+ {
+ "name": "HIP 106438",
+ "pos_x": -38.25,
+ "pos_y": -117.1875,
+ "pos_z": 102.3125,
+ "stations": "Bean Orbital,Smith Settlement"
+ },
+ {
+ "name": "HIP 106439",
+ "pos_x": -87.625,
+ "pos_y": -83.8125,
+ "pos_z": 67.6875,
+ "stations": "Langford Enterprise,MacLeod Landing"
+ },
+ {
+ "name": "HIP 106442",
+ "pos_x": 119.34375,
+ "pos_y": -127.8125,
+ "pos_z": 126.28125,
+ "stations": "Orban Hub"
+ },
+ {
+ "name": "HIP 106568",
+ "pos_x": -66.09375,
+ "pos_y": -128.78125,
+ "pos_z": 108.84375,
+ "stations": "Ptack Survey,Drake Dock,Wilhelm Orbital"
+ },
+ {
+ "name": "HIP 106586",
+ "pos_x": -22.46875,
+ "pos_y": -130.75,
+ "pos_z": 114.53125,
+ "stations": "Young Dock,Wetherill Station,Isaev Port,Webb Relay"
+ },
+ {
+ "name": "HIP 106591",
+ "pos_x": -57.3125,
+ "pos_y": -148.5,
+ "pos_z": 126.9375,
+ "stations": "Gunn's Progress,Wilhelm Survey"
+ },
+ {
+ "name": "HIP 106615",
+ "pos_x": 81.71875,
+ "pos_y": -184.5,
+ "pos_z": 172.34375,
+ "stations": "Theodor Winnecke Depot,Miller's Claim"
+ },
+ {
+ "name": "HIP 106700",
+ "pos_x": 46.5625,
+ "pos_y": -154.84375,
+ "pos_z": 141.6875,
+ "stations": "Kaneda Port,Lassell Terminal,Ings Vision"
+ },
+ {
+ "name": "HIP 106701",
+ "pos_x": -38.53125,
+ "pos_y": -151.375,
+ "pos_z": 129.8125,
+ "stations": "Shapiro Landing"
+ },
+ {
+ "name": "HIP 106707",
+ "pos_x": -136.78125,
+ "pos_y": -101.25,
+ "pos_z": 75.25,
+ "stations": "Brendan Port,Barentsz Platform,Minkowski Installation"
+ },
+ {
+ "name": "HIP 106714",
+ "pos_x": -20.53125,
+ "pos_y": -152.90625,
+ "pos_z": 132.84375,
+ "stations": "Jones Orbital,Gloss Camp,Carleson Point"
+ },
+ {
+ "name": "HIP 106749",
+ "pos_x": -43.5,
+ "pos_y": -133.21875,
+ "pos_z": 112.65625,
+ "stations": "Vaez de Torres Silo,Mikulin Station,Babcock Orbital,Havilland Colony"
+ },
+ {
+ "name": "HIP 106782",
+ "pos_x": 11.90625,
+ "pos_y": -130.8125,
+ "pos_z": 116.15625,
+ "stations": "Kerimov Colony,Crossfield Silo,Giacconi Laboratory,Naburimannu Port,Eggen Platform"
+ },
+ {
+ "name": "HIP 106792",
+ "pos_x": -42.46875,
+ "pos_y": -110.53125,
+ "pos_z": 92.46875,
+ "stations": "Halley Vision,Luiken Relay"
+ },
+ {
+ "name": "HIP 10682",
+ "pos_x": -92.4375,
+ "pos_y": -101.5,
+ "pos_z": -137.65625,
+ "stations": "Merle Settlement"
+ },
+ {
+ "name": "HIP 106868",
+ "pos_x": -65.59375,
+ "pos_y": -120.5625,
+ "pos_z": 98,
+ "stations": "Rice Keep"
+ },
+ {
+ "name": "HIP 106904",
+ "pos_x": 69.6875,
+ "pos_y": -164.96875,
+ "pos_z": 151.375,
+ "stations": "Niemeyer Depot"
+ },
+ {
+ "name": "HIP 10694",
+ "pos_x": 102.09375,
+ "pos_y": -160.3125,
+ "pos_z": 18.5625,
+ "stations": "Niemeyer Terminal,Horrocks Orbital,Gustafsson Hub,Pickering Dock,Hanke-Woods Orbital,Islam Orbital,Pollas Terminal,Bayer Orbital,Lunney Hub,Wachmann Enterprise,Ingstad Mine,Ashman Horizons"
+ },
+ {
+ "name": "HIP 106949",
+ "pos_x": -157.0625,
+ "pos_y": -61.90625,
+ "pos_z": 35.90625,
+ "stations": "Cochrane Prospect,Besonders Reach"
+ },
+ {
+ "name": "HIP 107001",
+ "pos_x": -95.9375,
+ "pos_y": -122.8125,
+ "pos_z": 95.0625,
+ "stations": "Pierres' Folly"
+ },
+ {
+ "name": "HIP 107010",
+ "pos_x": 76.25,
+ "pos_y": -96.53125,
+ "pos_z": 92.125,
+ "stations": "Baynes Terminal,Farghani Ring,Flammarion Dock,Porco Orbital,Baxter Relay,Herbert Reach,Lalande Dock,Cook Works,Mayer Enterprise,Watson Port"
+ },
+ {
+ "name": "HIP 107020",
+ "pos_x": -85.4375,
+ "pos_y": -83.375,
+ "pos_z": 61.96875,
+ "stations": "Winne Port"
+ },
+ {
+ "name": "HIP 107040",
+ "pos_x": -154.34375,
+ "pos_y": -50.78125,
+ "pos_z": 25.65625,
+ "stations": "Readdy Landing,Feynman Hub"
+ },
+ {
+ "name": "HIP 107042",
+ "pos_x": -31.5625,
+ "pos_y": -132.96875,
+ "pos_z": 110.78125,
+ "stations": "Zarnecki Relay,Giacobini Prospect"
+ },
+ {
+ "name": "HIP 10716",
+ "pos_x": 53.0625,
+ "pos_y": -226.1875,
+ "pos_z": -60.9375,
+ "stations": "Morgan Depot,van Royen Vision"
+ },
+ {
+ "name": "HIP 107172",
+ "pos_x": -11.59375,
+ "pos_y": -139.03125,
+ "pos_z": 116.875,
+ "stations": "Draper Orbital,Scithers Survey,Mukai Station,Napier Terminal"
+ },
+ {
+ "name": "HIP 107175",
+ "pos_x": -126.59375,
+ "pos_y": -64.90625,
+ "pos_z": 39.5625,
+ "stations": "Oltion Settlement"
+ },
+ {
+ "name": "HIP 107239",
+ "pos_x": 133.71875,
+ "pos_y": -103.34375,
+ "pos_z": 104.34375,
+ "stations": "Delsanti Port,Huxley Point,Newcomb Vision"
+ },
+ {
+ "name": "HIP 107295",
+ "pos_x": 14.3125,
+ "pos_y": -123.6875,
+ "pos_z": 106.0625,
+ "stations": "Wang Mine"
+ },
+ {
+ "name": "HIP 107299",
+ "pos_x": 40.09375,
+ "pos_y": -106.6875,
+ "pos_z": 95.03125,
+ "stations": "Bok Gateway,Bainbridge Terminal,Kraft Port,Bass Terminal,Turner Orbital,Thornycroft Exchange,Myasishchev Hub"
+ },
+ {
+ "name": "HIP 107314",
+ "pos_x": -134.75,
+ "pos_y": -52.78125,
+ "pos_z": 27,
+ "stations": "Lyell Station,Balandin Park"
+ },
+ {
+ "name": "HIP 107345",
+ "pos_x": 47.09375,
+ "pos_y": -99.8125,
+ "pos_z": 89.9375,
+ "stations": "Wigura City,Ambartsumian Dock,August von Steinheil Hub,Oberth Horizons"
+ },
+ {
+ "name": "HIP 107362",
+ "pos_x": 1.875,
+ "pos_y": -144.84375,
+ "pos_z": 121.6875,
+ "stations": "Ashman Platform,Howard Vision,Meuron Orbital"
+ },
+ {
+ "name": "HIP 107370",
+ "pos_x": -32.71875,
+ "pos_y": -163.78125,
+ "pos_z": 132.9375,
+ "stations": "Schilling Mines,Ziljak Landing"
+ },
+ {
+ "name": "HIP 107385",
+ "pos_x": 23.96875,
+ "pos_y": -94.3125,
+ "pos_z": 82.09375,
+ "stations": "Grzimek Vista,Winthrop Enterprise"
+ },
+ {
+ "name": "HIP 107395",
+ "pos_x": -105.28125,
+ "pos_y": -100.125,
+ "pos_z": 69.75,
+ "stations": "Bryusov Dock,Emshwiller Terminal,Carstensz Horizons"
+ },
+ {
+ "name": "HIP 107397",
+ "pos_x": 34.0625,
+ "pos_y": -165.5,
+ "pos_z": 142.875,
+ "stations": "Kaiser Vision"
+ },
+ {
+ "name": "HIP 107412",
+ "pos_x": -59.875,
+ "pos_y": -88.09375,
+ "pos_z": 65.5625,
+ "stations": "Kennan Stop,Bramah Dock,Jacquard Dock,Zamka Dock,Mendez Station,Gaspar de Lemos Legacy,Kerwin Enterprise,Vaez de Torres Point,Nolan Relay,Fidalgo Installation"
+ },
+ {
+ "name": "HIP 107476",
+ "pos_x": 34.53125,
+ "pos_y": -115.375,
+ "pos_z": 100.53125,
+ "stations": "Ayers Vision,Richer Port,Kosai Prospect,Tarter Bastion,Tapinas Enterprise"
+ },
+ {
+ "name": "HIP 107494",
+ "pos_x": -134.375,
+ "pos_y": -78.75,
+ "pos_z": 46.96875,
+ "stations": "Taylor Hub"
+ },
+ {
+ "name": "HIP 10754",
+ "pos_x": 162.21875,
+ "pos_y": -212,
+ "pos_z": 49.9375,
+ "stations": "Humason Enterprise,Mattingly Base,Youll Terminal"
+ },
+ {
+ "name": "HIP 107587",
+ "pos_x": -119.34375,
+ "pos_y": -91.34375,
+ "pos_z": 58.4375,
+ "stations": "Sellings Mines,Ray Station"
+ },
+ {
+ "name": "HIP 107591",
+ "pos_x": -126.21875,
+ "pos_y": -85.75,
+ "pos_z": 52.84375,
+ "stations": "Vercors Prospect"
+ },
+ {
+ "name": "HIP 107599",
+ "pos_x": -72.09375,
+ "pos_y": -144.21875,
+ "pos_z": 108.375,
+ "stations": "Galindo Freeport"
+ },
+ {
+ "name": "HIP 107614",
+ "pos_x": 55.84375,
+ "pos_y": -101.3125,
+ "pos_z": 91.125,
+ "stations": "Parker Platform,Rice Bastion"
+ },
+ {
+ "name": "HIP 107618",
+ "pos_x": -69.0625,
+ "pos_y": -117.78125,
+ "pos_z": 86.9375,
+ "stations": "Roberts Ring,al-Khayyam Survey,Efremov Installation"
+ },
+ {
+ "name": "HIP 107779",
+ "pos_x": -8.75,
+ "pos_y": -139.125,
+ "pos_z": 111.21875,
+ "stations": "Ulloa Relay,Robert Mine"
+ },
+ {
+ "name": "HIP 10779",
+ "pos_x": 116,
+ "pos_y": -188.1875,
+ "pos_z": 17.25,
+ "stations": "Penn Camp,Siddha Terminal,Chadwick Terminal"
+ },
+ {
+ "name": "HIP 107805",
+ "pos_x": -53,
+ "pos_y": -91.90625,
+ "pos_z": 66.21875,
+ "stations": "McCarthy Enterprise,Walker Silo"
+ },
+ {
+ "name": "HIP 107806",
+ "pos_x": 38.40625,
+ "pos_y": -96.46875,
+ "pos_z": 83.65625,
+ "stations": "Zhuravleva Terminal"
+ },
+ {
+ "name": "HIP 107821",
+ "pos_x": -152.875,
+ "pos_y": -54.4375,
+ "pos_z": 20.75,
+ "stations": "Quaglia Ring,Krupkat Enterprise,Kippax Mines,Palmer Camp,Vinge Hub"
+ },
+ {
+ "name": "HIP 107847",
+ "pos_x": 129.625,
+ "pos_y": -136.875,
+ "pos_z": 129.78125,
+ "stations": "van der Riet Woolley Beacon,Ellison Silo"
+ },
+ {
+ "name": "HIP 10786",
+ "pos_x": 147.5,
+ "pos_y": -162.59375,
+ "pos_z": 60.125,
+ "stations": "Reinhold Hub,Oberth Gateway,Weill Palace,Zhukovsky Station,Penzias Hub,Hereford Orbital,Nobel Enterprise,Jakes Enterprise,Donati Port,Austin Port,Gelfand Silo,Valz Colony"
+ },
+ {
+ "name": "HIP 107936",
+ "pos_x": 7.84375,
+ "pos_y": -97.78125,
+ "pos_z": 79.28125,
+ "stations": "Lane Hub,Kotelnikov Orbital,Schulhof Terminal"
+ },
+ {
+ "name": "HIP 107985",
+ "pos_x": -46.6875,
+ "pos_y": -137.59375,
+ "pos_z": 102.0625,
+ "stations": "Fischer Refinery"
+ },
+ {
+ "name": "HIP 107994",
+ "pos_x": 37.5625,
+ "pos_y": -164.0625,
+ "pos_z": 136.28125,
+ "stations": "Dishoeck Vision,Samos Mines"
+ },
+ {
+ "name": "HIP 108041",
+ "pos_x": 83.96875,
+ "pos_y": -98.25,
+ "pos_z": 91.1875,
+ "stations": "Bahcall Mines,Meinel Mines"
+ },
+ {
+ "name": "HIP 108070",
+ "pos_x": 31.65625,
+ "pos_y": -180.125,
+ "pos_z": 147.28125,
+ "stations": "Sandage Station,Longomontanus Enterprise"
+ },
+ {
+ "name": "HIP 108095",
+ "pos_x": -71.40625,
+ "pos_y": -131.53125,
+ "pos_z": 91.9375,
+ "stations": "Bolotov City,Wilson Terminal,Bluford Terminal,Pierres Enterprise"
+ },
+ {
+ "name": "HIP 108110",
+ "pos_x": -122.625,
+ "pos_y": 22,
+ "pos_z": -37.34375,
+ "stations": "Coppel Depot,Trevithick Enterprise,Papin Relay"
+ },
+ {
+ "name": "HIP 108154",
+ "pos_x": -177.03125,
+ "pos_y": -40.03125,
+ "pos_z": 2.1875,
+ "stations": "Cook's Claim"
+ },
+ {
+ "name": "HIP 108158",
+ "pos_x": 67.96875,
+ "pos_y": -80.59375,
+ "pos_z": 74.34375,
+ "stations": "Alexeyev Terminal,Norton Installation,Schachner Vision"
+ },
+ {
+ "name": "HIP 10818",
+ "pos_x": -17.375,
+ "pos_y": -104.4375,
+ "pos_z": -69.125,
+ "stations": "Kiernan Terminal,Bunnell Horizons,Herbert Vision,Ing Base"
+ },
+ {
+ "name": "HIP 108228",
+ "pos_x": -122.09375,
+ "pos_y": -85.25,
+ "pos_z": 46,
+ "stations": "Ramaswamy Orbital"
+ },
+ {
+ "name": "HIP 108256",
+ "pos_x": 99.65625,
+ "pos_y": -133.71875,
+ "pos_z": 120.78125,
+ "stations": "Pacheco Hub,Tago Orbital,Kohoutek Terminal"
+ },
+ {
+ "name": "HIP 108258",
+ "pos_x": 18.8125,
+ "pos_y": -151,
+ "pos_z": 120.59375,
+ "stations": "Wolfe Point,Frazetta Point,Beer Port"
+ },
+ {
+ "name": "HIP 108282",
+ "pos_x": -159.84375,
+ "pos_y": -80.53125,
+ "pos_z": 35.3125,
+ "stations": "Bass Installation,Pohl Relay"
+ },
+ {
+ "name": "HIP 108288",
+ "pos_x": 74.46875,
+ "pos_y": -176.0625,
+ "pos_z": 149.1875,
+ "stations": "Freas Depot,Hertzsprung Hub"
+ },
+ {
+ "name": "HIP 108322",
+ "pos_x": 160.59375,
+ "pos_y": -126.90625,
+ "pos_z": 125.65625,
+ "stations": "Tevis Installation"
+ },
+ {
+ "name": "HIP 10834",
+ "pos_x": 137.65625,
+ "pos_y": -185.375,
+ "pos_z": 38.90625,
+ "stations": "Brom Orbital,van Royen Station,de Kamp Dock,Bounds Prospect,William Sargent Orbital,Hoffmeister Dock,Barnard Dock,Brunner Hub,Hind Reach,Wrangel Enterprise,Peary Prospect"
+ },
+ {
+ "name": "HIP 108344",
+ "pos_x": 56.375,
+ "pos_y": -132.4375,
+ "pos_z": 111.90625,
+ "stations": "Cyrrhus Station,Palisa Hub,Kelly Orbital,Lindemann Port,Debehogne Vision,van de Sande Bakhuyzen Dock,Kamov Station,Spielberg Laboratory,Gotlieb Lab,Moriarty Penal colony"
+ },
+ {
+ "name": "HIP 108375",
+ "pos_x": -9.09375,
+ "pos_y": -119.03125,
+ "pos_z": 90.09375,
+ "stations": "Libeskind Port"
+ },
+ {
+ "name": "HIP 108419",
+ "pos_x": -54.625,
+ "pos_y": -105.375,
+ "pos_z": 71.28125,
+ "stations": "Norman Base,Banks Mine"
+ },
+ {
+ "name": "HIP 108422",
+ "pos_x": 84.96875,
+ "pos_y": -126.6875,
+ "pos_z": 112.09375,
+ "stations": "Fraknoi Reach,Crumey Installation,Bridger Barracks"
+ },
+ {
+ "name": "HIP 108438",
+ "pos_x": -106.0625,
+ "pos_y": -125.71875,
+ "pos_z": 77.75,
+ "stations": "Elvstrom Holdings"
+ },
+ {
+ "name": "HIP 108464",
+ "pos_x": 98.65625,
+ "pos_y": -166.75,
+ "pos_z": 144.9375,
+ "stations": "Esclangon Point,Bohme Mine"
+ },
+ {
+ "name": "HIP 108589",
+ "pos_x": 7.3125,
+ "pos_y": -147.84375,
+ "pos_z": 113.25,
+ "stations": "Phillips Landing,Armero Vision"
+ },
+ {
+ "name": "HIP 108598",
+ "pos_x": 34.09375,
+ "pos_y": -111.96875,
+ "pos_z": 90.875,
+ "stations": "Dillon Dock,Secchi Vision,Holden Point,Korolev Dock,Dumont Dock,Hagihara Vision,Oort Vision,Koishikawa Station,Brundage Vision,Jung Base,Chaffee Station,Sutter Hub,Jansky Mine"
+ },
+ {
+ "name": "HIP 108602",
+ "pos_x": -154.375,
+ "pos_y": -1.96875,
+ "pos_z": -26.65625,
+ "stations": "Zamyatin Barracks"
+ },
+ {
+ "name": "HIP 108701",
+ "pos_x": 35.875,
+ "pos_y": -151.96875,
+ "pos_z": 120.625,
+ "stations": "Kagawa Ring,MacCready Dock,Schmitz Arsenal,Theodorsen Prospect"
+ },
+ {
+ "name": "HIP 108729",
+ "pos_x": 38.5625,
+ "pos_y": -146.3125,
+ "pos_z": 116.6875,
+ "stations": "Bailly Station,Shinjo Port,Elmore Port,Arfstrom Terminal,Bolton Orbital"
+ },
+ {
+ "name": "HIP 108806",
+ "pos_x": -120.625,
+ "pos_y": -86.125,
+ "pos_z": 41.0625,
+ "stations": "Kregel Orbital,Gubarev Station,Copernicus Colony,Bujold Penal colony,Metz Survey"
+ },
+ {
+ "name": "HIP 108820",
+ "pos_x": -98.0625,
+ "pos_y": -77.09375,
+ "pos_z": 38.46875,
+ "stations": "Hernandez Works,Creighton's Exile"
+ },
+ {
+ "name": "HIP 108822",
+ "pos_x": -114.78125,
+ "pos_y": -80.125,
+ "pos_z": 37.5,
+ "stations": "Newcomen Depot,Fujimori Arena"
+ },
+ {
+ "name": "HIP 108859",
+ "pos_x": -138.5625,
+ "pos_y": -77.8125,
+ "pos_z": 30.90625,
+ "stations": "Nehsi Dock,White Terminal"
+ },
+ {
+ "name": "HIP 108912",
+ "pos_x": 44.09375,
+ "pos_y": -98.34375,
+ "pos_z": 81,
+ "stations": "Kuo Station,Schumacher Penal colony,Alden Terminal"
+ },
+ {
+ "name": "HIP 108923",
+ "pos_x": -44.8125,
+ "pos_y": -129.5,
+ "pos_z": 86.59375,
+ "stations": "Mitchell Sanctuary"
+ },
+ {
+ "name": "HIP 108950",
+ "pos_x": -6.25,
+ "pos_y": -133.125,
+ "pos_z": 96.40625,
+ "stations": "McDermott Vision,Birkhoff Beacon,Thorne Hangar"
+ },
+ {
+ "name": "HIP 108971",
+ "pos_x": 51.71875,
+ "pos_y": -150.34375,
+ "pos_z": 120.28125,
+ "stations": "Yerka Platform,Babakin Station,Crossfield Hub,Frimout Horizons"
+ },
+ {
+ "name": "HIP 108996",
+ "pos_x": -55.625,
+ "pos_y": -150.84375,
+ "pos_z": 99.3125,
+ "stations": "Hawker Terminal"
+ },
+ {
+ "name": "HIP 109012",
+ "pos_x": -16.0625,
+ "pos_y": -129.125,
+ "pos_z": 91.03125,
+ "stations": "Impey Hub,Cellarius Terminal,Ocampo Horizons,Schuster Terminal"
+ },
+ {
+ "name": "HIP 109058",
+ "pos_x": -84.5,
+ "pos_y": -122.5625,
+ "pos_z": 72.25,
+ "stations": "Dalmas Point"
+ },
+ {
+ "name": "HIP 10906",
+ "pos_x": 113.75,
+ "pos_y": -228.59375,
+ "pos_z": -6.875,
+ "stations": "Delporte Settlement,Biruni Platform,Martin Works"
+ },
+ {
+ "name": "HIP 109067",
+ "pos_x": -131,
+ "pos_y": -92.125,
+ "pos_z": 40.75,
+ "stations": "Hume Orbital,Brooks Survey,Conway Terminal,Varthema Vision,Linaweaver Park"
+ },
+ {
+ "name": "HIP 109144",
+ "pos_x": -112.1875,
+ "pos_y": -110.09375,
+ "pos_z": 56.75,
+ "stations": "Willis Ring,Ross City,Bass Enterprise"
+ },
+ {
+ "name": "HIP 109182",
+ "pos_x": -181.25,
+ "pos_y": -56.09375,
+ "pos_z": 3.3125,
+ "stations": "George Vision"
+ },
+ {
+ "name": "HIP 109203",
+ "pos_x": 64.9375,
+ "pos_y": -136.3125,
+ "pos_z": 111.25,
+ "stations": "Sweet City,Hawkes Depot,Alexandria's Inheritance"
+ },
+ {
+ "name": "HIP 109228",
+ "pos_x": 61.40625,
+ "pos_y": -146.6875,
+ "pos_z": 117.8125,
+ "stations": "Adkins Orbital,Allen City,Sheremetevsky Beacon,Urkovic Lab,Alexander Relay"
+ },
+ {
+ "name": "HIP 109310",
+ "pos_x": -161,
+ "pos_y": -38.5625,
+ "pos_z": -6.375,
+ "stations": "Lanier Silo,Fremion Settlement,Westerfeld Landing"
+ },
+ {
+ "name": "HIP 109330",
+ "pos_x": -68.46875,
+ "pos_y": -139.9375,
+ "pos_z": 84.96875,
+ "stations": "Fujikawa Base"
+ },
+ {
+ "name": "HIP 109368",
+ "pos_x": 110.71875,
+ "pos_y": -106.34375,
+ "pos_z": 98.71875,
+ "stations": "Leonard Works"
+ },
+ {
+ "name": "HIP 109381",
+ "pos_x": 0.71875,
+ "pos_y": -113.125,
+ "pos_z": 80.125,
+ "stations": "Robert Barracks,Stephan City"
+ },
+ {
+ "name": "HIP 10941",
+ "pos_x": 169.65625,
+ "pos_y": -178.03125,
+ "pos_z": 72.5625,
+ "stations": "Chongzhi Port,Myasishchev Port,Sikorsky Dock,Anthony Plant"
+ },
+ {
+ "name": "HIP 109423",
+ "pos_x": -42.90625,
+ "pos_y": -155.875,
+ "pos_z": 100.59375,
+ "stations": "Rolland Ring,Great's Folly,Fernandes de Queiros Terminal,Barbaro Hub,Chwedyk Holdings"
+ },
+ {
+ "name": "HIP 109462",
+ "pos_x": -26.59375,
+ "pos_y": -181.1875,
+ "pos_z": 121.53125,
+ "stations": "Oja Platform,Dunlop Landing,Chern Arsenal"
+ },
+ {
+ "name": "HIP 109468",
+ "pos_x": -1.3125,
+ "pos_y": -130.03125,
+ "pos_z": 91,
+ "stations": "Finlay-Freundlich Horizons,Albategnius Horizons,Houten-Groeneveld Beacon,Kosai Refinery"
+ },
+ {
+ "name": "HIP 109479",
+ "pos_x": -127.5,
+ "pos_y": 29.875,
+ "pos_z": -48.5,
+ "stations": "Wul Orbital,Kandel City,Gold Prospect,Burgess Station"
+ },
+ {
+ "name": "HIP 109490",
+ "pos_x": -120.875,
+ "pos_y": -78.625,
+ "pos_z": 28.9375,
+ "stations": "Grant Mines,Horowitz Observatory"
+ },
+ {
+ "name": "HIP 109528",
+ "pos_x": -60.6875,
+ "pos_y": -101.1875,
+ "pos_z": 57.53125,
+ "stations": "Cartan Dock,Heyerdahl Dock,Doyle Dock,Arnason Holdings"
+ },
+ {
+ "name": "HIP 109549",
+ "pos_x": -19.0625,
+ "pos_y": -155.21875,
+ "pos_z": 104.15625,
+ "stations": "Nagata Gateway,Graham Port,Phillips Settlement"
+ },
+ {
+ "name": "HIP 10961",
+ "pos_x": -138.40625,
+ "pos_y": -20.03125,
+ "pos_z": -144.375,
+ "stations": "Aldiss Penal colony,Russo Installation"
+ },
+ {
+ "name": "HIP 109612",
+ "pos_x": 38.5625,
+ "pos_y": -123.78125,
+ "pos_z": 94.375,
+ "stations": "Yakovlev Vision,Mustelin Terminal,Stephan Terminal,Cassini Settlement,Melotte Base,Nakamura Dock"
+ },
+ {
+ "name": "HIP 109642",
+ "pos_x": 52.125,
+ "pos_y": -176.5,
+ "pos_z": 133.65625,
+ "stations": "Mayor's Claim,Charlois Arsenal"
+ },
+ {
+ "name": "HIP 10973",
+ "pos_x": 57.21875,
+ "pos_y": -209.125,
+ "pos_z": -52.6875,
+ "stations": "Jackson Mine"
+ },
+ {
+ "name": "HIP 109782",
+ "pos_x": 13.3125,
+ "pos_y": -160.5625,
+ "pos_z": 112.875,
+ "stations": "Bisnovatyi-Kogan Vision,Chacornac Holdings,Proctor Terminal,Thorne Vision"
+ },
+ {
+ "name": "HIP 109787",
+ "pos_x": 42.53125,
+ "pos_y": -156.0625,
+ "pos_z": 116.34375,
+ "stations": "Tsiolkovsky Platform,Nomen Station,Spassky Survey,Gann Refinery"
+ },
+ {
+ "name": "HIP 109918",
+ "pos_x": -58.15625,
+ "pos_y": -124.25,
+ "pos_z": 70.625,
+ "stations": "Sleator Dock,Plucker's Progress,Agrippa Base"
+ },
+ {
+ "name": "HIP 109956",
+ "pos_x": 50.46875,
+ "pos_y": -181.125,
+ "pos_z": 133.90625,
+ "stations": "Grushin Prospect,Potagos Base"
+ },
+ {
+ "name": "HIP 110",
+ "pos_x": -137.96875,
+ "pos_y": -60.84375,
+ "pos_z": -58.9375,
+ "stations": "Bachman Orbital,Coles Hub"
+ },
+ {
+ "name": "HIP 110014",
+ "pos_x": -62.34375,
+ "pos_y": -114.875,
+ "pos_z": 62.40625,
+ "stations": "Bose Dock,Broglie Colony"
+ },
+ {
+ "name": "HIP 110028",
+ "pos_x": -39.3125,
+ "pos_y": -183.84375,
+ "pos_z": 113.875,
+ "stations": "Zander Dock,Moresby Base,Hampson Relay"
+ },
+ {
+ "name": "HIP 110084",
+ "pos_x": -20.4375,
+ "pos_y": -123.40625,
+ "pos_z": 77.3125,
+ "stations": "Allen Orbital,Mather Base,Vavrova Survey"
+ },
+ {
+ "name": "HIP 110094",
+ "pos_x": -188.59375,
+ "pos_y": 12.5625,
+ "pos_z": -53.3125,
+ "stations": "Ostrander Colony,Chasles Platform"
+ },
+ {
+ "name": "HIP 110102",
+ "pos_x": 84.15625,
+ "pos_y": -164.1875,
+ "pos_z": 129.1875,
+ "stations": "Jansky Works"
+ },
+ {
+ "name": "HIP 110105",
+ "pos_x": 140.0625,
+ "pos_y": -177,
+ "pos_z": 151.03125,
+ "stations": "Schoening's Claim"
+ },
+ {
+ "name": "HIP 110115",
+ "pos_x": -7.5,
+ "pos_y": -198.21875,
+ "pos_z": 129.78125,
+ "stations": "Montanari Base,West Mines,Tank Vision"
+ },
+ {
+ "name": "HIP 110127",
+ "pos_x": -4.65625,
+ "pos_y": -154.90625,
+ "pos_z": 101.625,
+ "stations": "Hereford Point,Rich Base"
+ },
+ {
+ "name": "HIP 110147",
+ "pos_x": 29.03125,
+ "pos_y": -147.375,
+ "pos_z": 104.5625,
+ "stations": "Alexandria Gateway,Israel Port,Wilson Dock,Lloyd Wright Terminal,Skiff Port,Mueller Gateway,Powers' Inheritance,van der Riet Woolley Prospect,Evangelisti Works,Lukyanenko Installation,Richer City"
+ },
+ {
+ "name": "HIP 110156",
+ "pos_x": -23.53125,
+ "pos_y": -107.125,
+ "pos_z": 65.21875,
+ "stations": "Kirshner Vision,Giles Keep"
+ },
+ {
+ "name": "HIP 110205",
+ "pos_x": -107.90625,
+ "pos_y": -81.25,
+ "pos_z": 27.34375,
+ "stations": "Tokubei Hub"
+ },
+ {
+ "name": "HIP 110219",
+ "pos_x": -59.8125,
+ "pos_y": -121.9375,
+ "pos_z": 65.6875,
+ "stations": "Garnier Mine,Dedman Settlement,Kennicott Prospect,Canty Point"
+ },
+ {
+ "name": "HIP 110245",
+ "pos_x": -6.78125,
+ "pos_y": -101.625,
+ "pos_z": 65.0625,
+ "stations": "Penn Silo,Vogel Station,Fox Survey"
+ },
+ {
+ "name": "HIP 110248",
+ "pos_x": 130.65625,
+ "pos_y": -139.125,
+ "pos_z": 123.125,
+ "stations": "Glass Landing,Xin Station,Carstensz Bastion"
+ },
+ {
+ "name": "HIP 110262",
+ "pos_x": -24.78125,
+ "pos_y": -178.65625,
+ "pos_z": 111.03125,
+ "stations": "Pittendreigh Platform,Gilliland Base,Shinjo Colony"
+ },
+ {
+ "name": "HIP 110291",
+ "pos_x": -186.8125,
+ "pos_y": -29.5,
+ "pos_z": -26.53125,
+ "stations": "Clough Sanctuary"
+ },
+ {
+ "name": "HIP 110309",
+ "pos_x": -152.4375,
+ "pos_y": -4.9375,
+ "pos_z": -34.25,
+ "stations": "Yolen Dock,Gerrold Prospect,Haber Holdings"
+ },
+ {
+ "name": "HIP 110337",
+ "pos_x": 4.5,
+ "pos_y": -122.90625,
+ "pos_z": 81.21875,
+ "stations": "Adelman City,Fowler Platform"
+ },
+ {
+ "name": "HIP 110339",
+ "pos_x": -154.5625,
+ "pos_y": -25.375,
+ "pos_z": -21.59375,
+ "stations": "So-yeon Enterprise,Ericsson Platform,Hinz Observatory"
+ },
+ {
+ "name": "HIP 110355",
+ "pos_x": 83.875,
+ "pos_y": -167.84375,
+ "pos_z": 129.9375,
+ "stations": "Iwamoto Platform,Salgari Depot"
+ },
+ {
+ "name": "HIP 110376",
+ "pos_x": 55.6875,
+ "pos_y": -168.46875,
+ "pos_z": 123.1875,
+ "stations": "Binney Mines,Ferguson Dock,Arnold Schwassmann Hub"
+ },
+ {
+ "name": "HIP 110397",
+ "pos_x": 146.875,
+ "pos_y": -99.4375,
+ "pos_z": 100.96875,
+ "stations": "Hamuy Port,Haro Dock,Abell Hub,Tilman Installation"
+ },
+ {
+ "name": "HIP 110424",
+ "pos_x": -74.84375,
+ "pos_y": -175.5625,
+ "pos_z": 94.71875,
+ "stations": "Smith Installation"
+ },
+ {
+ "name": "HIP 110440",
+ "pos_x": -1.9375,
+ "pos_y": -148.21875,
+ "pos_z": 95.125,
+ "stations": "Russell Oasis,Arfstrom Prospect,Wilhelm von Struve Prospect"
+ },
+ {
+ "name": "HIP 110442",
+ "pos_x": 68.25,
+ "pos_y": -152.84375,
+ "pos_z": 115.6875,
+ "stations": "Bowell Horizons,Prandtl Dock,Arp Station,Kent's Inheritance,Utkin Colony"
+ },
+ {
+ "name": "HIP 110483",
+ "pos_x": -23.46875,
+ "pos_y": -108.375,
+ "pos_z": 63.6875,
+ "stations": "Valigursky Orbital,Struzan Dock,Gold Hub,Hansen Orbital,Wolf Orbital,Bigourdan Hub,Takahashi Relay,Heck Landing,Hussey Landing,Shapiro Base"
+ },
+ {
+ "name": "HIP 110512",
+ "pos_x": -42.625,
+ "pos_y": -146.03125,
+ "pos_z": 82.6875,
+ "stations": "Santarem Terminal,Jolliet Terminal,Langford Enterprise"
+ },
+ {
+ "name": "HIP 110564",
+ "pos_x": 102.25,
+ "pos_y": -164.8125,
+ "pos_z": 131.125,
+ "stations": "Kanai Enterprise"
+ },
+ {
+ "name": "HIP 110626",
+ "pos_x": -154.4375,
+ "pos_y": -42.0625,
+ "pos_z": -13.1875,
+ "stations": "Yolen Landing"
+ },
+ {
+ "name": "HIP 110629",
+ "pos_x": 2.75,
+ "pos_y": -125.875,
+ "pos_z": 80.4375,
+ "stations": "Finlay Vision,Miklouho-Maclay Enterprise,Banach Barracks"
+ },
+ {
+ "name": "HIP 110632",
+ "pos_x": 4.3125,
+ "pos_y": -196.71875,
+ "pos_z": 125.6875,
+ "stations": "Louis de Lacaille Depot,Binney Mines"
+ },
+ {
+ "name": "HIP 110692",
+ "pos_x": -12.5,
+ "pos_y": -115.71875,
+ "pos_z": 69.5,
+ "stations": "Shepherd Hub,Alexander Gateway,Kerr Terminal,Burton Base,Rothman Vision"
+ },
+ {
+ "name": "HIP 110695",
+ "pos_x": -36.125,
+ "pos_y": -116.53125,
+ "pos_z": 63.8125,
+ "stations": "Crown Beacon,Dupuy de Lome Arena,McAllaster Beacon"
+ },
+ {
+ "name": "HIP 110753",
+ "pos_x": -90.125,
+ "pos_y": -112.625,
+ "pos_z": 46.8125,
+ "stations": "Ferguson Enterprise,Tanner Port"
+ },
+ {
+ "name": "HIP 110773",
+ "pos_x": -120.375,
+ "pos_y": 46.375,
+ "pos_z": -60.6875,
+ "stations": "Lounge Ring,Citroen Ring,Sarich Orbital,Shiras Settlement"
+ },
+ {
+ "name": "HIP 110776",
+ "pos_x": -106.875,
+ "pos_y": -105.90625,
+ "pos_z": 37.96875,
+ "stations": "Puleston Dock,Godwin Survey,Bradbury Dock,Fermat Dock"
+ },
+ {
+ "name": "HIP 110797",
+ "pos_x": 38.34375,
+ "pos_y": -124.5,
+ "pos_z": 87.6875,
+ "stations": "Hottot Horizons,Dyson Prospect,Merrill Terminal"
+ },
+ {
+ "name": "HIP 110852",
+ "pos_x": 106.34375,
+ "pos_y": -108.1875,
+ "pos_z": 95.375,
+ "stations": "Zhukovsky Dock"
+ },
+ {
+ "name": "HIP 110867",
+ "pos_x": 143.4375,
+ "pos_y": -103.96875,
+ "pos_z": 102.625,
+ "stations": "Schulhof Platform,Ziemianski Installation,Tanaka Point"
+ },
+ {
+ "name": "HIP 110887",
+ "pos_x": 45.8125,
+ "pos_y": -118.375,
+ "pos_z": 85.40625,
+ "stations": "Antonov Port,Brosnatch Port,Gehrels Vision,Tolstoi Bastion,Lindblad Lab"
+ },
+ {
+ "name": "HIP 110892",
+ "pos_x": -14.59375,
+ "pos_y": -153.1875,
+ "pos_z": 90.6875,
+ "stations": "Lane Landing,Boyajian Observatory"
+ },
+ {
+ "name": "HIP 110943",
+ "pos_x": -140.4375,
+ "pos_y": -107.5625,
+ "pos_z": 28.1875,
+ "stations": "Noether Enterprise"
+ },
+ {
+ "name": "HIP 110952",
+ "pos_x": 36.15625,
+ "pos_y": -193.40625,
+ "pos_z": 128.5,
+ "stations": "Melia Gateway,Ackerman Vista"
+ },
+ {
+ "name": "HIP 110954",
+ "pos_x": 24.5625,
+ "pos_y": -126.71875,
+ "pos_z": 84.40625,
+ "stations": "Tank Port,Tucker Lab,Lindblad Terminal"
+ },
+ {
+ "name": "HIP 110987",
+ "pos_x": -11.6875,
+ "pos_y": -185.46875,
+ "pos_z": 110.375,
+ "stations": "Brashear Oasis,Bennett Landing"
+ },
+ {
+ "name": "HIP 111027",
+ "pos_x": 34.78125,
+ "pos_y": -202.75,
+ "pos_z": 133.1875,
+ "stations": "Dalgarno Settlement,Clark Base,Littrow Terminal,Francisco de Eliza Laboratory"
+ },
+ {
+ "name": "HIP 111096",
+ "pos_x": 151.125,
+ "pos_y": -160.5,
+ "pos_z": 138.8125,
+ "stations": "Stebbins Terminal"
+ },
+ {
+ "name": "HIP 11111",
+ "pos_x": -92.4375,
+ "pos_y": 21.3125,
+ "pos_z": -78.96875,
+ "stations": "Hadfield Survey,Coney Installation"
+ },
+ {
+ "name": "HIP 111153",
+ "pos_x": 135.3125,
+ "pos_y": -122.3125,
+ "pos_z": 111.15625,
+ "stations": "Stromgren Dock,Corben Terminal,Dilworth City,Nourse Point"
+ },
+ {
+ "name": "HIP 111173",
+ "pos_x": -85.53125,
+ "pos_y": -137.625,
+ "pos_z": 59.125,
+ "stations": "Lagrange Station,Leichhardt Port"
+ },
+ {
+ "name": "HIP 11125",
+ "pos_x": 37.78125,
+ "pos_y": -145.84375,
+ "pos_z": -40.4375,
+ "stations": "Brooks Vision,Marriott Mines,al-Khayyam Survey,Kelly Vision,Brill Hub"
+ },
+ {
+ "name": "HIP 111272",
+ "pos_x": 73.5,
+ "pos_y": -182.03125,
+ "pos_z": 129.15625,
+ "stations": "Kondo Depot,Schommer Stop,Rondon Installation"
+ },
+ {
+ "name": "HIP 111285",
+ "pos_x": 41.4375,
+ "pos_y": -197.5,
+ "pos_z": 129.28125,
+ "stations": "Harrington Terminal,Raleigh Vision"
+ },
+ {
+ "name": "HIP 11131",
+ "pos_x": 32.15625,
+ "pos_y": -119,
+ "pos_z": -31.71875,
+ "stations": "Sawyer Beacon,Verne Vision,Vries Terminal"
+ },
+ {
+ "name": "HIP 111369",
+ "pos_x": -155.21875,
+ "pos_y": -83.71875,
+ "pos_z": 5.34375,
+ "stations": "Cole Station,Sargent Mine"
+ },
+ {
+ "name": "HIP 1114",
+ "pos_x": 82.40625,
+ "pos_y": -191.6875,
+ "pos_z": 75.5,
+ "stations": "Alphonsi Gateway,Shinjo Enterprise,Searle Hub,Messerschmitt Terminal,Apianus Port,Norman Sanctuary"
+ },
+ {
+ "name": "HIP 11144",
+ "pos_x": 98.65625,
+ "pos_y": -260.21875,
+ "pos_z": -41.90625,
+ "stations": "Ortiz Moreno Base,Arago Prospect"
+ },
+ {
+ "name": "HIP 111447",
+ "pos_x": 18.6875,
+ "pos_y": -198.65625,
+ "pos_z": 121.875,
+ "stations": "Biesbroeck's Claim"
+ },
+ {
+ "name": "HIP 111478",
+ "pos_x": 79.3125,
+ "pos_y": -100.1875,
+ "pos_z": 81.375,
+ "stations": "Kelly Hub,Xuesen Market"
+ },
+ {
+ "name": "HIP 111486",
+ "pos_x": 133.25,
+ "pos_y": -131.15625,
+ "pos_z": 115,
+ "stations": "Fischer Ring,Leuschner Port,Gurevich Vision,Naubakht Station,Hanke-Woods Terminal,Ikeya Gateway,Cady Hub"
+ },
+ {
+ "name": "HIP 111496",
+ "pos_x": -136.75,
+ "pos_y": 13.71875,
+ "pos_z": -47.53125,
+ "stations": "Searfoss City,Barsanti Legacy"
+ },
+ {
+ "name": "HIP 11157",
+ "pos_x": -68.1875,
+ "pos_y": -110.4375,
+ "pos_z": -125.59375,
+ "stations": "Roberts Orbital,Dias Orbital,Meredith Survey"
+ },
+ {
+ "name": "HIP 11160",
+ "pos_x": 133.375,
+ "pos_y": -207.96875,
+ "pos_z": 19.65625,
+ "stations": "Antonov Vision"
+ },
+ {
+ "name": "HIP 111616",
+ "pos_x": 10.96875,
+ "pos_y": -152,
+ "pos_z": 90.90625,
+ "stations": "Shimizu Orbital,Stith Terminal,Bassford Silo"
+ },
+ {
+ "name": "HIP 111640",
+ "pos_x": -57.46875,
+ "pos_y": -141.75,
+ "pos_z": 64.75,
+ "stations": "Sturgeon Point,Stein Colony,Buffett Point"
+ },
+ {
+ "name": "HIP 111657",
+ "pos_x": -103.9375,
+ "pos_y": -86.4375,
+ "pos_z": 19.125,
+ "stations": "Narvaez's Claim"
+ },
+ {
+ "name": "HIP 111672",
+ "pos_x": 18.1875,
+ "pos_y": -151.15625,
+ "pos_z": 92.09375,
+ "stations": "Panshin Landing,Wallis Colony"
+ },
+ {
+ "name": "HIP 111698",
+ "pos_x": -25.6875,
+ "pos_y": -114.59375,
+ "pos_z": 58.03125,
+ "stations": "Ambartsumian Vision,Andrews Depot,Ziegler Refinery,Hildebrandt Hub,Mayor Holdings"
+ },
+ {
+ "name": "HIP 111707",
+ "pos_x": 125.625,
+ "pos_y": -153,
+ "pos_z": 124.6875,
+ "stations": "Banneker Station"
+ },
+ {
+ "name": "HIP 111755",
+ "pos_x": 10.25,
+ "pos_y": -117.9375,
+ "pos_z": 70.1875,
+ "stations": "Mooz Station,Kohoutek Vision,Sheffield Horizons,Taylor Jr. Port,Sutter Port,Oxley Base,Fuller Vision"
+ },
+ {
+ "name": "HIP 111808",
+ "pos_x": -111.21875,
+ "pos_y": -115.34375,
+ "pos_z": 32.1875,
+ "stations": "Eschbach Port,Carver Platform"
+ },
+ {
+ "name": "HIP 11182",
+ "pos_x": -25.75,
+ "pos_y": -100.875,
+ "pos_z": -79.375,
+ "stations": "Herreshoff Market,Barnes Enterprise"
+ },
+ {
+ "name": "HIP 111880",
+ "pos_x": -16.5625,
+ "pos_y": -113.96875,
+ "pos_z": 59.1875,
+ "stations": "Slusser Installation,Hoshide City"
+ },
+ {
+ "name": "HIP 111910",
+ "pos_x": -84.9375,
+ "pos_y": -142.09375,
+ "pos_z": 54.0625,
+ "stations": "Tuan Platform,Lee Beacon"
+ },
+ {
+ "name": "HIP 11192",
+ "pos_x": 16.375,
+ "pos_y": -185.5,
+ "pos_z": -83.625,
+ "stations": "Kawasato Port,Keldysh Landing,Giancola Station,Moffat Survey,Wallis Penal colony,Derleth Colony"
+ },
+ {
+ "name": "HIP 112001",
+ "pos_x": 8.34375,
+ "pos_y": -236.3125,
+ "pos_z": 134.0625,
+ "stations": "Gaughan Dock,Sagan City,Mather Dock"
+ },
+ {
+ "name": "HIP 112002",
+ "pos_x": 78.34375,
+ "pos_y": -135.4375,
+ "pos_z": 99.28125,
+ "stations": "Schommer Ring,Bothezat Vision,Martinez Bastion,Altshuller Prospect,Kojima Vision"
+ },
+ {
+ "name": "HIP 112013",
+ "pos_x": -0.6875,
+ "pos_y": -150.15625,
+ "pos_z": 83.21875,
+ "stations": "Schwabe Hub,Darwin Horizons,Wild Installation"
+ },
+ {
+ "name": "HIP 112042",
+ "pos_x": -172.34375,
+ "pos_y": -38.78125,
+ "pos_z": -31.375,
+ "stations": "Pryor Base"
+ },
+ {
+ "name": "HIP 112049",
+ "pos_x": 95.53125,
+ "pos_y": -77.5,
+ "pos_z": 72.25,
+ "stations": "Adams Vision,Dingle Palace,Ball Silo"
+ },
+ {
+ "name": "HIP 11205",
+ "pos_x": 59.90625,
+ "pos_y": -162.875,
+ "pos_z": -28.8125,
+ "stations": "Niemeyer Vision"
+ },
+ {
+ "name": "HIP 112052",
+ "pos_x": -0.3125,
+ "pos_y": -155.375,
+ "pos_z": 85.9375,
+ "stations": "Shalatula's Folly,Witt Platform,Sei Hub"
+ },
+ {
+ "name": "HIP 112069",
+ "pos_x": -36.25,
+ "pos_y": -106.9375,
+ "pos_z": 47.96875,
+ "stations": "Morrill Point,Tisserand Stop"
+ },
+ {
+ "name": "HIP 112095",
+ "pos_x": -67.03125,
+ "pos_y": -99.5625,
+ "pos_z": 34.21875,
+ "stations": "Savitskaya City"
+ },
+ {
+ "name": "HIP 112113",
+ "pos_x": 83.03125,
+ "pos_y": -102.0625,
+ "pos_z": 81.8125,
+ "stations": "Nomen Vision,Roth Ring,Uto Enterprise,Aitken Relay,Romer City"
+ },
+ {
+ "name": "HIP 112123",
+ "pos_x": 15.71875,
+ "pos_y": -220.28125,
+ "pos_z": 125.9375,
+ "stations": "De Platform,Kraft Survey,Hoerner Colony,Piercy Depot"
+ },
+ {
+ "name": "HIP 112135",
+ "pos_x": -72.21875,
+ "pos_y": -104.90625,
+ "pos_z": 35.15625,
+ "stations": "Song Dock"
+ },
+ {
+ "name": "HIP 11218",
+ "pos_x": -16.78125,
+ "pos_y": -151.71875,
+ "pos_z": -98.25,
+ "stations": "North Ring,Silverberg Prospect,Pellegrino Base"
+ },
+ {
+ "name": "HIP 112201",
+ "pos_x": 72.65625,
+ "pos_y": -130.09375,
+ "pos_z": 93.6875,
+ "stations": "McCool Vision,Silves Survey,Babakin Terminal,Nahavandi Dock"
+ },
+ {
+ "name": "HIP 11222",
+ "pos_x": 80.5,
+ "pos_y": -214.96875,
+ "pos_z": -36.84375,
+ "stations": "Delaunay Mines,van den Hove Dock,Clark Base"
+ },
+ {
+ "name": "HIP 112240",
+ "pos_x": -132.59375,
+ "pos_y": -133.4375,
+ "pos_z": 30.90625,
+ "stations": "Gromov Horizons"
+ },
+ {
+ "name": "HIP 112268",
+ "pos_x": -135.25,
+ "pos_y": -98.03125,
+ "pos_z": 10.5625,
+ "stations": "Boole Beacon,Schouten Relay"
+ },
+ {
+ "name": "HIP 112315",
+ "pos_x": 62.75,
+ "pos_y": -175.78125,
+ "pos_z": 114.625,
+ "stations": "Beg Port,Potez Hub,Stephens Point,Sugano Terminal"
+ },
+ {
+ "name": "HIP 112368",
+ "pos_x": -176.84375,
+ "pos_y": -82.96875,
+ "pos_z": -11.6875,
+ "stations": "Katzenstein Landing,Mitchison Base,Brule Depot"
+ },
+ {
+ "name": "HIP 112389",
+ "pos_x": -106.3125,
+ "pos_y": -73.90625,
+ "pos_z": 5.71875,
+ "stations": "Morgan Penal colony,Mitchell Depot,Fozard Point"
+ },
+ {
+ "name": "HIP 112400",
+ "pos_x": -45.65625,
+ "pos_y": -123.25,
+ "pos_z": 51.3125,
+ "stations": "Gunn Prospect,Bluford Orbital,Springer Colony"
+ },
+ {
+ "name": "HIP 11241",
+ "pos_x": 30.5625,
+ "pos_y": -214.53125,
+ "pos_z": -86.0625,
+ "stations": "Esposito City,Paczynski Station,Celsius Terminal,Vuia Settlement,Cousin Terminal"
+ },
+ {
+ "name": "HIP 112441",
+ "pos_x": 40.65625,
+ "pos_y": -114.46875,
+ "pos_z": 73.90625,
+ "stations": "Baracchi Hub,Cleve Installation"
+ },
+ {
+ "name": "HIP 112462",
+ "pos_x": -56.46875,
+ "pos_y": -162.5,
+ "pos_z": 68.125,
+ "stations": "Grigorovich Survey,Lyot Vision,Digges Terminal,Lewis Point,Kepler's Inheritance"
+ },
+ {
+ "name": "HIP 112494",
+ "pos_x": -109.09375,
+ "pos_y": -149.71875,
+ "pos_z": 44.0625,
+ "stations": "Beliaev Port,Effinger Stop,Judson Survey"
+ },
+ {
+ "name": "HIP 112506",
+ "pos_x": -101.625,
+ "pos_y": -74.0625,
+ "pos_z": 6.34375,
+ "stations": "Antonelli Terminal,Glidden Colony,Gerrold Installation,Rushd Enterprise"
+ },
+ {
+ "name": "HIP 112512",
+ "pos_x": -52.34375,
+ "pos_y": -127.84375,
+ "pos_z": 50.625,
+ "stations": "Foden Colony,Sladek Port,Tilman Installation"
+ },
+ {
+ "name": "HIP 112521",
+ "pos_x": -120.3125,
+ "pos_y": -126.15625,
+ "pos_z": 27.71875,
+ "stations": "Banach Mines,Hassanein Reformatory"
+ },
+ {
+ "name": "HIP 112522",
+ "pos_x": -106.75,
+ "pos_y": -124.90625,
+ "pos_z": 31.4375,
+ "stations": "Gibson Freeport"
+ },
+ {
+ "name": "HIP 112535",
+ "pos_x": -134.46875,
+ "pos_y": -92,
+ "pos_z": 5.03125,
+ "stations": "Auer City,Darboux Gateway,Ramon Gateway,Antonio de Andrade Port"
+ },
+ {
+ "name": "HIP 112549",
+ "pos_x": 63.28125,
+ "pos_y": -205.0625,
+ "pos_z": 128.5,
+ "stations": "Fowler Colony,Baille Terminal,Clement Settlement"
+ },
+ {
+ "name": "HIP 112580",
+ "pos_x": -135.125,
+ "pos_y": -114.53125,
+ "pos_z": 16.25,
+ "stations": "Heinlein Dock,Potagos Installation,Fozard Barracks"
+ },
+ {
+ "name": "HIP 11263",
+ "pos_x": 104.96875,
+ "pos_y": -168.65625,
+ "pos_z": 11.75,
+ "stations": "Hay Port,Wilhelm Klinkerfues Ring,Froud Station"
+ },
+ {
+ "name": "HIP 112643",
+ "pos_x": -34.9375,
+ "pos_y": -148.5,
+ "pos_z": 66.0625,
+ "stations": "Baille's Claim"
+ },
+ {
+ "name": "HIP 112648",
+ "pos_x": -24.4375,
+ "pos_y": -126.8125,
+ "pos_z": 58.15625,
+ "stations": "Riebe Gateway,Francisco de Eliza Survey"
+ },
+ {
+ "name": "HIP 112663",
+ "pos_x": 20.8125,
+ "pos_y": -199.28125,
+ "pos_z": 110.6875,
+ "stations": "Uto Colony,Leopold Heckmann Station,Hammel Prospect,Chilton Base"
+ },
+ {
+ "name": "HIP 11273",
+ "pos_x": -86.4375,
+ "pos_y": -22.78125,
+ "pos_z": -97.65625,
+ "stations": "Cernan Platform,Seega Colony"
+ },
+ {
+ "name": "HIP 112753",
+ "pos_x": 80.65625,
+ "pos_y": -136.125,
+ "pos_z": 96.90625,
+ "stations": "Wales Port"
+ },
+ {
+ "name": "HIP 112823",
+ "pos_x": -138.375,
+ "pos_y": -52.78125,
+ "pos_z": -18.96875,
+ "stations": "Markov Reformatory,Tanaka Mine"
+ },
+ {
+ "name": "HIP 112829",
+ "pos_x": -130.40625,
+ "pos_y": -10.875,
+ "pos_z": -37.84375,
+ "stations": "MacKellar Hub,Melroy Plant,Barr Survey"
+ },
+ {
+ "name": "HIP 112859",
+ "pos_x": 135.75,
+ "pos_y": -106.78125,
+ "pos_z": 99.8125,
+ "stations": "Fujikawa Colony,Poyser Orbital,Ballard Beacon,Stokes Arsenal"
+ },
+ {
+ "name": "HIP 112908",
+ "pos_x": 19.875,
+ "pos_y": -132.8125,
+ "pos_z": 74.1875,
+ "stations": "Reber Vision"
+ },
+ {
+ "name": "HIP 112913",
+ "pos_x": 41.71875,
+ "pos_y": -147.3125,
+ "pos_z": 88.78125,
+ "stations": "Chelomey Orbital,Kimura Port,Schomburg Silo,Apianus Lab"
+ },
+ {
+ "name": "HIP 112932",
+ "pos_x": -140.6875,
+ "pos_y": -131.4375,
+ "pos_z": 19.1875,
+ "stations": "Barreiros Survey,Alexandrov's Claim"
+ },
+ {
+ "name": "HIP 112970",
+ "pos_x": -176.25,
+ "pos_y": 26.125,
+ "pos_z": -72.6875,
+ "stations": "Lee Holdings,Stjepan Seljan Dock,McMahon's Folly,Hughes Vision"
+ },
+ {
+ "name": "HIP 112974",
+ "pos_x": -59.09375,
+ "pos_y": -107.875,
+ "pos_z": 34.4375,
+ "stations": "Bachman Port,Skolem Hub,la Cosa City,Eskridge Port,West Station,Palmer Hub,Mitchell Prospect,Islam Survey,Siegel Depot"
+ },
+ {
+ "name": "HIP 113010",
+ "pos_x": -2.625,
+ "pos_y": -129.03125,
+ "pos_z": 63.9375,
+ "stations": "Hamuy Hub"
+ },
+ {
+ "name": "HIP 113019",
+ "pos_x": 102.375,
+ "pos_y": -192.5625,
+ "pos_z": 131.40625,
+ "stations": "Orban Survey"
+ },
+ {
+ "name": "HIP 113040",
+ "pos_x": 21.375,
+ "pos_y": -188.3125,
+ "pos_z": 101.5625,
+ "stations": "Dreyer Mines,van den Bergh Dock,Silva Bastion"
+ },
+ {
+ "name": "HIP 11305",
+ "pos_x": 43.4375,
+ "pos_y": -163.5625,
+ "pos_z": -46.65625,
+ "stations": "Shirazi Terminal,Rizvi Dock,Luu Platform"
+ },
+ {
+ "name": "HIP 113076",
+ "pos_x": -127.28125,
+ "pos_y": -53.59375,
+ "pos_z": -16.71875,
+ "stations": "Heisenberg Terminal,Barry Station,Archer Terminal"
+ },
+ {
+ "name": "HIP 113086",
+ "pos_x": -115.5625,
+ "pos_y": -81.34375,
+ "pos_z": 1.0625,
+ "stations": "Flynn Relay,Junlong Landing"
+ },
+ {
+ "name": "HIP 113100",
+ "pos_x": 18.65625,
+ "pos_y": -139.3125,
+ "pos_z": 75.71875,
+ "stations": "Pu Station,McMillan Vision"
+ },
+ {
+ "name": "HIP 113103",
+ "pos_x": 9.6875,
+ "pos_y": -123.78125,
+ "pos_z": 64.90625,
+ "stations": "Mattei Station,Whitney Keep,Salam Dock"
+ },
+ {
+ "name": "HIP 113113",
+ "pos_x": 80.96875,
+ "pos_y": -142.90625,
+ "pos_z": 98.75,
+ "stations": "McDermott Mines,Christopher Installation"
+ },
+ {
+ "name": "HIP 113126",
+ "pos_x": 11,
+ "pos_y": -196.4375,
+ "pos_z": 101.28125,
+ "stations": "Giger Orbital,Brom Station,Holden Base,Nelder Relay"
+ },
+ {
+ "name": "HIP 113128",
+ "pos_x": -59.21875,
+ "pos_y": -152.375,
+ "pos_z": 55.3125,
+ "stations": "Friedman Installation,Norman Hub"
+ },
+ {
+ "name": "HIP 113142",
+ "pos_x": 30.65625,
+ "pos_y": -211.84375,
+ "pos_z": 115.53125,
+ "stations": "Bulychev Horizons,Whitney Orbital,Wilhelm von Struve Holdings"
+ },
+ {
+ "name": "HIP 113219",
+ "pos_x": 42.90625,
+ "pos_y": -186.15625,
+ "pos_z": 106.28125,
+ "stations": "Krenkel Keep,Romer Terminal"
+ },
+ {
+ "name": "HIP 113250",
+ "pos_x": -71.53125,
+ "pos_y": -148.96875,
+ "pos_z": 48.21875,
+ "stations": "Hillary Settlement,Lee Relay"
+ },
+ {
+ "name": "HIP 113293",
+ "pos_x": 135.25,
+ "pos_y": -144.09375,
+ "pos_z": 117.34375,
+ "stations": "Maskelyne Depot,Garden Bastion"
+ },
+ {
+ "name": "HIP 113355",
+ "pos_x": -81.8125,
+ "pos_y": -119.4375,
+ "pos_z": 29.09375,
+ "stations": "McDivitt Terminal,Burke Bastion,Atwater Gateway,Behring Vision,Gibson Works"
+ },
+ {
+ "name": "HIP 113386",
+ "pos_x": -41.46875,
+ "pos_y": -181.75,
+ "pos_z": 73.0625,
+ "stations": "Godel Beacon,Daqing Terminal,Harrington Prospect"
+ },
+ {
+ "name": "HIP 1134",
+ "pos_x": -56.4375,
+ "pos_y": -142.78125,
+ "pos_z": -9.125,
+ "stations": "Lassell Dock,de Andrade Keep,Naylor's Pride,McCrea Prospect"
+ },
+ {
+ "name": "HIP 113402",
+ "pos_x": 80.875,
+ "pos_y": -193.9375,
+ "pos_z": 121.78125,
+ "stations": "Bok Vision,Somayaji Terminal,Bertin Settlement"
+ },
+ {
+ "name": "HIP 113422",
+ "pos_x": 18.59375,
+ "pos_y": -169.21875,
+ "pos_z": 87.78125,
+ "stations": "Bouvard Survey,Donaldson Depot,van der Riet Woolley Survey,Rawat Reach"
+ },
+ {
+ "name": "HIP 113423",
+ "pos_x": 6.5625,
+ "pos_y": -109.1875,
+ "pos_z": 54.71875,
+ "stations": "Marsden Depot,Dingle Survey"
+ },
+ {
+ "name": "HIP 113430",
+ "pos_x": 82.625,
+ "pos_y": -143.75,
+ "pos_z": 98.09375,
+ "stations": "Kapteyn Gateway,Check Vision,Hickam Terminal,Baille Dock,Kamov Terminal,Opik Gateway,Fiorilla Keep,Helffrich Vision,Kepler Port"
+ },
+ {
+ "name": "HIP 113454",
+ "pos_x": 17.125,
+ "pos_y": -131.4375,
+ "pos_z": 68.9375,
+ "stations": "Kuo Hub,Ziegler Vision,Delporte Holdings"
+ },
+ {
+ "name": "HIP 113477",
+ "pos_x": -121.40625,
+ "pos_y": 19.78125,
+ "pos_z": -52.46875,
+ "stations": "Papin Platform,Cartwright Relay,Egan Vision"
+ },
+ {
+ "name": "HIP 113495",
+ "pos_x": -104.90625,
+ "pos_y": -86.40625,
+ "pos_z": 3.90625,
+ "stations": "Thoreau Station,Horowitz Port,Sharma Terminal,O'Brien's Inheritance"
+ },
+ {
+ "name": "HIP 11352",
+ "pos_x": -70.6875,
+ "pos_y": -54.59375,
+ "pos_z": -100.15625,
+ "stations": "Hire Gateway,Tognini Ring,Evans Ring,Cleave Terminal,Macan Landing,Sylvester Settlement,Brash Terminal,Alexandria Orbital,Grunsfeld Port,Binet City,Hopkinson Enterprise,Resnick Penal colony,Scott Terminal"
+ },
+ {
+ "name": "HIP 113528",
+ "pos_x": -20.40625,
+ "pos_y": -148.15625,
+ "pos_z": 63,
+ "stations": "Vogel Station,Herschel Dock"
+ },
+ {
+ "name": "HIP 113535",
+ "pos_x": -138.8125,
+ "pos_y": -43.375,
+ "pos_z": -28.9375,
+ "stations": "Bertin Settlement"
+ },
+ {
+ "name": "HIP 113543",
+ "pos_x": -66.4375,
+ "pos_y": -164.34375,
+ "pos_z": 54.09375,
+ "stations": "Helffrich Survey,Sy's Claim,Haber Lab,Silverberg Bastion"
+ },
+ {
+ "name": "HIP 11359",
+ "pos_x": 29.125,
+ "pos_y": -145.9375,
+ "pos_z": -51.8125,
+ "stations": "Orbik Port,Leonard Bastion,Kimberlin Laboratory"
+ },
+ {
+ "name": "HIP 113598",
+ "pos_x": -16.90625,
+ "pos_y": -196.09375,
+ "pos_z": 86.1875,
+ "stations": "Rees Prospect"
+ },
+ {
+ "name": "HIP 11360",
+ "pos_x": -30.75,
+ "pos_y": -111.21875,
+ "pos_z": -91.96875,
+ "stations": "DeLucas Settlement,Noakes Settlement,Coke Survey"
+ },
+ {
+ "name": "HIP 113607",
+ "pos_x": 47.4375,
+ "pos_y": -177.34375,
+ "pos_z": 100.34375,
+ "stations": "Whitcomb Survey,Reed Laboratory,Powell Barracks"
+ },
+ {
+ "name": "HIP 113632",
+ "pos_x": 3.84375,
+ "pos_y": -145.625,
+ "pos_z": 69.65625,
+ "stations": "Eisinga Terminal,Maire Keep,Delsanti's Claim,Pelt Horizons"
+ },
+ {
+ "name": "HIP 113699",
+ "pos_x": -83.375,
+ "pos_y": -97.25,
+ "pos_z": 15.0625,
+ "stations": "Bond Terminal,Weston Dock,Binnie Outpost"
+ },
+ {
+ "name": "HIP 113712",
+ "pos_x": -111.25,
+ "pos_y": -127.03125,
+ "pos_z": 18.6875,
+ "stations": "Kozeyev Vision"
+ },
+ {
+ "name": "HIP 113760",
+ "pos_x": 10.09375,
+ "pos_y": -118.625,
+ "pos_z": 58.53125,
+ "stations": "Whitney Relay,Theodor Winnecke Base"
+ },
+ {
+ "name": "HIP 113807",
+ "pos_x": 133.46875,
+ "pos_y": -112.0625,
+ "pos_z": 100.28125,
+ "stations": "Zel'dovich Station,Strzelecki Observatory,Hatanaka Orbital"
+ },
+ {
+ "name": "HIP 113812",
+ "pos_x": 0.09375,
+ "pos_y": -163.34375,
+ "pos_z": 75.15625,
+ "stations": "Encke Dock,Bertin Bastion"
+ },
+ {
+ "name": "HIP 113839",
+ "pos_x": 26.5,
+ "pos_y": -157.6875,
+ "pos_z": 82,
+ "stations": "Kirby Landing"
+ },
+ {
+ "name": "HIP 113842",
+ "pos_x": 58.6875,
+ "pos_y": -177.34375,
+ "pos_z": 102.71875,
+ "stations": "Austin Hub,Szentmartony Base,Chiang Survey"
+ },
+ {
+ "name": "HIP 113858",
+ "pos_x": 16.3125,
+ "pos_y": -184.375,
+ "pos_z": 90.25,
+ "stations": "Marley Depot,Sabine Settlement,Darwin Vision"
+ },
+ {
+ "name": "HIP 113894",
+ "pos_x": -109.53125,
+ "pos_y": -106.34375,
+ "pos_z": 8.09375,
+ "stations": "Cheli Survey,Otto Base,Pierce Enterprise"
+ },
+ {
+ "name": "HIP 113921",
+ "pos_x": 71.0625,
+ "pos_y": -216.53125,
+ "pos_z": 124.53125,
+ "stations": "Frost Terminal,Jewitt Terminal,Ortiz Moreno Landing"
+ },
+ {
+ "name": "HIP 113942",
+ "pos_x": 7.625,
+ "pos_y": -219.5625,
+ "pos_z": 102.28125,
+ "stations": "Naubakht Colony,Sargent Vision,Merle Installation"
+ },
+ {
+ "name": "HIP 113948",
+ "pos_x": -38.0625,
+ "pos_y": -169.9375,
+ "pos_z": 62.8125,
+ "stations": "Baliunas Port,Anastase Perrotin Hub,Wallis Vision,Wilson Port,Hawkes Installation,Fidalgo Penal colony,Weyn Enterprise,Dhawan Vision"
+ },
+ {
+ "name": "HIP 113980",
+ "pos_x": -93.09375,
+ "pos_y": -111,
+ "pos_z": 15.46875,
+ "stations": "Grimwood Gateway,Clapperton Station,Belyanin Ring,Dantec Station,Pippin Terminal,Williams Depot"
+ },
+ {
+ "name": "HIP 114040",
+ "pos_x": 32.25,
+ "pos_y": -176.875,
+ "pos_z": 91.1875,
+ "stations": "Bolton Gateway,Antoniadi Survey,Nagata Beacon,Chandra Installation"
+ },
+ {
+ "name": "HIP 114044",
+ "pos_x": -7.78125,
+ "pos_y": -114.84375,
+ "pos_z": 48.46875,
+ "stations": "Gurney Terminal,Ikeya Landing,Milne Relay,Fan Ring,Whelan Survey"
+ },
+ {
+ "name": "HIP 114091",
+ "pos_x": 82.34375,
+ "pos_y": -214.15625,
+ "pos_z": 126,
+ "stations": "Kibalchich Platform,Koolhaas Port,Kalam Arsenal"
+ },
+ {
+ "name": "HIP 114099",
+ "pos_x": -153.28125,
+ "pos_y": 26.65625,
+ "pos_z": -69.34375,
+ "stations": "Davy Survey,Underwood Mines,Shatalov Mines"
+ },
+ {
+ "name": "HIP 114135",
+ "pos_x": -35.75,
+ "pos_y": -120.84375,
+ "pos_z": 40,
+ "stations": "Timofeyevich Point,Lockwood Prospect,Dixon Hub"
+ },
+ {
+ "name": "HIP 11417",
+ "pos_x": -30.21875,
+ "pos_y": -142.5,
+ "pos_z": -109.84375,
+ "stations": "Strzelecki Point"
+ },
+ {
+ "name": "HIP 114188",
+ "pos_x": 70.3125,
+ "pos_y": -200.78125,
+ "pos_z": 114.875,
+ "stations": "Sewell Survey,Tanaka Terminal"
+ },
+ {
+ "name": "HIP 114195",
+ "pos_x": 23.25,
+ "pos_y": -214.53125,
+ "pos_z": 103.03125,
+ "stations": "Bradley Works"
+ },
+ {
+ "name": "HIP 114236",
+ "pos_x": 75.46875,
+ "pos_y": -142,
+ "pos_z": 90.625,
+ "stations": "Bohr Platform"
+ },
+ {
+ "name": "HIP 114269",
+ "pos_x": 81.15625,
+ "pos_y": -150.84375,
+ "pos_z": 96.46875,
+ "stations": "Martiniere Outpost"
+ },
+ {
+ "name": "HIP 114291",
+ "pos_x": -24.9375,
+ "pos_y": -116.5,
+ "pos_z": 41.03125,
+ "stations": "Karlsefni Settlement"
+ },
+ {
+ "name": "HIP 114328",
+ "pos_x": 11.53125,
+ "pos_y": -206.1875,
+ "pos_z": 93.46875,
+ "stations": "Zarnecki Hub,Ziewe Hub"
+ },
+ {
+ "name": "HIP 11433",
+ "pos_x": 31.09375,
+ "pos_y": -122.6875,
+ "pos_z": -37.9375,
+ "stations": "Impey Landing,Dawes Prospect,Searle Platform,Ikeya Survey,Muhammad Ibn Battuta Horizons"
+ },
+ {
+ "name": "HIP 114333",
+ "pos_x": 94.1875,
+ "pos_y": -224.25,
+ "pos_z": 132.8125,
+ "stations": "Sweet Orbital,Bolkow Orbital,Swift Silo,Mallett Prospect"
+ },
+ {
+ "name": "HIP 114340",
+ "pos_x": -68.375,
+ "pos_y": -150.53125,
+ "pos_z": 38.71875,
+ "stations": "Oxley Station,Pacheco Hub"
+ },
+ {
+ "name": "HIP 114367",
+ "pos_x": 80.78125,
+ "pos_y": -125.03125,
+ "pos_z": 84.75,
+ "stations": "Borrego Orbital,Shipton Terminal"
+ },
+ {
+ "name": "HIP 114420",
+ "pos_x": -139.375,
+ "pos_y": -26.90625,
+ "pos_z": -42.1875,
+ "stations": "Vasilyev Gateway,Schade Station,Eilenberg Landing,Spielberg Terminal,Auld Survey"
+ },
+ {
+ "name": "HIP 114422",
+ "pos_x": 19.34375,
+ "pos_y": -234.8125,
+ "pos_z": 107.75,
+ "stations": "McIntosh Dock,Banks Depot"
+ },
+ {
+ "name": "HIP 114443",
+ "pos_x": 18.5,
+ "pos_y": -131.8125,
+ "pos_z": 63.28125,
+ "stations": "Baracchi Colony"
+ },
+ {
+ "name": "HIP 114460",
+ "pos_x": 71.625,
+ "pos_y": -105.375,
+ "pos_z": 72.46875,
+ "stations": "Yuzhe Survey,Leberecht Tempel Escape"
+ },
+ {
+ "name": "HIP 114472",
+ "pos_x": 78.09375,
+ "pos_y": -242.40625,
+ "pos_z": 133.125,
+ "stations": "Check's Claim,Edwards Vista,Tombaugh's Claim"
+ },
+ {
+ "name": "HIP 114477",
+ "pos_x": 35.96875,
+ "pos_y": -145,
+ "pos_z": 75.4375,
+ "stations": "Chernykh Station"
+ },
+ {
+ "name": "HIP 11448",
+ "pos_x": 52.25,
+ "pos_y": -154.78125,
+ "pos_z": -35.09375,
+ "stations": "Cottenot Orbital,Arnason Base"
+ },
+ {
+ "name": "HIP 114503",
+ "pos_x": 75.25,
+ "pos_y": -128.25,
+ "pos_z": 83.40625,
+ "stations": "Hoyle Works"
+ },
+ {
+ "name": "HIP 114512",
+ "pos_x": -140.4375,
+ "pos_y": -32,
+ "pos_z": -41.09375,
+ "stations": "Fernao do Po Settlement,Barnes Prospect"
+ },
+ {
+ "name": "HIP 114519",
+ "pos_x": -56.1875,
+ "pos_y": -152.40625,
+ "pos_z": 42.375,
+ "stations": "Yakovlev Orbital,Barbuy Station,Gurevich Vision,Metz Prospect,Tavernier Depot"
+ },
+ {
+ "name": "HIP 114530",
+ "pos_x": 19.5625,
+ "pos_y": -144.4375,
+ "pos_z": 68.375,
+ "stations": "Hansen City,Perlmutter Vision"
+ },
+ {
+ "name": "HIP 114585",
+ "pos_x": 50.40625,
+ "pos_y": -177.375,
+ "pos_z": 93.6875,
+ "stations": "Goldberg Dock,Finney Base,Argelander Port,Lopez-Garcia Vision,Nearchus Holdings"
+ },
+ {
+ "name": "HIP 114588",
+ "pos_x": -104.3125,
+ "pos_y": -119.90625,
+ "pos_z": 9.0625,
+ "stations": "Jahn Beacon,Haberlandt Depot,Hermite's Inheritance"
+ },
+ {
+ "name": "HIP 11459",
+ "pos_x": 98.25,
+ "pos_y": -258.5625,
+ "pos_z": -47.90625,
+ "stations": "Hevelius' Claim,Brendan Point"
+ },
+ {
+ "name": "HIP 114614",
+ "pos_x": 36.9375,
+ "pos_y": -178.53125,
+ "pos_z": 88.625,
+ "stations": "Bailly's Claim"
+ },
+ {
+ "name": "HIP 114638",
+ "pos_x": 101.6875,
+ "pos_y": -223.21875,
+ "pos_z": 132.46875,
+ "stations": "Sheremetevsky Survey"
+ },
+ {
+ "name": "HIP 114683",
+ "pos_x": -144.71875,
+ "pos_y": -113.28125,
+ "pos_z": -10.5,
+ "stations": "Forstchen Oasis,Grimwood Colony"
+ },
+ {
+ "name": "HIP 11469",
+ "pos_x": 166.34375,
+ "pos_y": -239.28125,
+ "pos_z": 30.84375,
+ "stations": "Gaughan Station,Pogson Settlement,Safdie Survey,Medupe Installation,Tanner Prospect"
+ },
+ {
+ "name": "HIP 1147",
+ "pos_x": -29.53125,
+ "pos_y": -178.25,
+ "pos_z": 11.125,
+ "stations": "Bayer Port"
+ },
+ {
+ "name": "HIP 114702",
+ "pos_x": -57.34375,
+ "pos_y": -111.40625,
+ "pos_z": 23.125,
+ "stations": "Thagard Gateway,Artyukhin Port,Bowen Gateway,Bernoulli Orbital,Detmer Ring,Thuot City"
+ },
+ {
+ "name": "HIP 114709",
+ "pos_x": 51.71875,
+ "pos_y": -190.1875,
+ "pos_z": 98.59375,
+ "stations": "McKean City,Nakamura Station,Bolton Dock,Verrier Vision,Sheremetevsky Terminal,Hafner Terminal,Roche Orbital,Smyth Station,Fowler Hub,Gentil Settlement,Watts Port"
+ },
+ {
+ "name": "HIP 114736",
+ "pos_x": 43.5625,
+ "pos_y": -139.59375,
+ "pos_z": 74.40625,
+ "stations": "Deutsch Colony,Fairey Dock,Schwabe Terminal"
+ },
+ {
+ "name": "HIP 114743",
+ "pos_x": 11.4375,
+ "pos_y": -219.125,
+ "pos_z": 94.15625,
+ "stations": "Powers Port"
+ },
+ {
+ "name": "HIP 114746",
+ "pos_x": -15.4375,
+ "pos_y": -112.96875,
+ "pos_z": 40.0625,
+ "stations": "Niijima Survey"
+ },
+ {
+ "name": "HIP 114878",
+ "pos_x": -1.21875,
+ "pos_y": -240.71875,
+ "pos_z": 96.375,
+ "stations": "Agrippa Terminal,Peral Silo,Moffat Vision,Mozhaysky Colony"
+ },
+ {
+ "name": "HIP 114880",
+ "pos_x": 68.65625,
+ "pos_y": -155.5625,
+ "pos_z": 90.09375,
+ "stations": "Langford Installation,Verne Dock,Burckhardt Dock,Mitchell Terminal,Franke Horizons,Fernao do Po Port,Vonarburg Station,Christopher Orbital,Anvil Hub,Artin Enterprise,Smirnova Base"
+ },
+ {
+ "name": "HIP 11493",
+ "pos_x": 105,
+ "pos_y": -230.53125,
+ "pos_z": -25.875,
+ "stations": "Arai Settlement,Moore Landing,Euthymenes' Folly"
+ },
+ {
+ "name": "HIP 114952",
+ "pos_x": -62.96875,
+ "pos_y": -116.0625,
+ "pos_z": 20.90625,
+ "stations": "Satcher Settlement"
+ },
+ {
+ "name": "HIP 114960",
+ "pos_x": -101.46875,
+ "pos_y": -176.46875,
+ "pos_z": 29.40625,
+ "stations": "Blaylock Retreat"
+ },
+ {
+ "name": "HIP 114967",
+ "pos_x": 26.21875,
+ "pos_y": -173.875,
+ "pos_z": 79.8125,
+ "stations": "van den Hove Platform"
+ },
+ {
+ "name": "HIP 115094",
+ "pos_x": -7.8125,
+ "pos_y": -196.65625,
+ "pos_z": 73.78125,
+ "stations": "Parsons Prospect,Stiles Station,Frechet's Claim"
+ },
+ {
+ "name": "HIP 115151",
+ "pos_x": 112.03125,
+ "pos_y": -155.59375,
+ "pos_z": 106.25,
+ "stations": "McKee Base,Nakamura Vision,Antonov Enterprise"
+ },
+ {
+ "name": "HIP 115181",
+ "pos_x": 57.90625,
+ "pos_y": -174.5,
+ "pos_z": 91.25,
+ "stations": "Biermann Terminal,Hirayama Vision,Stone Hub,Daniel Base"
+ },
+ {
+ "name": "HIP 115220",
+ "pos_x": -81.21875,
+ "pos_y": -135.375,
+ "pos_z": 18.65625,
+ "stations": "Bartoe Terminal,Eskridge Dock,Schachner Settlement"
+ },
+ {
+ "name": "HIP 115247",
+ "pos_x": 113.34375,
+ "pos_y": -126.65625,
+ "pos_z": 95.28125,
+ "stations": "Rees Enterprise,Fabricius Depot"
+ },
+ {
+ "name": "HIP 115275",
+ "pos_x": 34.46875,
+ "pos_y": -166.9375,
+ "pos_z": 78.03125,
+ "stations": "Longomontanus Colony,Arp Dock,Clement Escape,Barnwell Colony"
+ },
+ {
+ "name": "HIP 115277",
+ "pos_x": -119.09375,
+ "pos_y": -44.40625,
+ "pos_z": -32.25,
+ "stations": "Dirac Mine,Smith Base"
+ },
+ {
+ "name": "HIP 115314",
+ "pos_x": -23.1875,
+ "pos_y": -209,
+ "pos_z": 69.875,
+ "stations": "Ing Camp,Darwin Horizons,Zenbei Vision"
+ },
+ {
+ "name": "HIP 115328",
+ "pos_x": 53.25,
+ "pos_y": -176.78125,
+ "pos_z": 89.125,
+ "stations": "Tomita Ring,Denning City,Barcelos' Inheritance,Vavrova Hub"
+ },
+ {
+ "name": "HIP 115337",
+ "pos_x": 18.8125,
+ "pos_y": -257.4375,
+ "pos_z": 105.3125,
+ "stations": "Sitterly Dock,Connes Bastion"
+ },
+ {
+ "name": "HIP 115361",
+ "pos_x": 38.75,
+ "pos_y": -217.1875,
+ "pos_z": 98.03125,
+ "stations": "Valigursky Orbital,Bouwens Vision,Lundmark Terminal,Hottot Silo,Hodgkinson's Progress"
+ },
+ {
+ "name": "HIP 11537",
+ "pos_x": 55.25,
+ "pos_y": -180.96875,
+ "pos_z": -48.03125,
+ "stations": "Amano Dock"
+ },
+ {
+ "name": "HIP 115370",
+ "pos_x": -126.15625,
+ "pos_y": -101.71875,
+ "pos_z": -14.3125,
+ "stations": "Apt Stop"
+ },
+ {
+ "name": "HIP 115380",
+ "pos_x": 80.9375,
+ "pos_y": -190.0625,
+ "pos_z": 105.15625,
+ "stations": "Schoenherr Dock"
+ },
+ {
+ "name": "HIP 115386",
+ "pos_x": 33.28125,
+ "pos_y": -191.9375,
+ "pos_z": 85.9375,
+ "stations": "Naubakht Colony"
+ },
+ {
+ "name": "HIP 115411",
+ "pos_x": -39.84375,
+ "pos_y": -134.75,
+ "pos_z": 33.75,
+ "stations": "Wisdom Prospect,Mitchison Vision"
+ },
+ {
+ "name": "HIP 115417",
+ "pos_x": -95.71875,
+ "pos_y": -74.59375,
+ "pos_z": -12.15625,
+ "stations": "Jendrassik Dock,Grissom Landing"
+ },
+ {
+ "name": "HIP 115473",
+ "pos_x": -131.78125,
+ "pos_y": -35.25,
+ "pos_z": -42.3125,
+ "stations": "Al Saud Dock,Laplace Landing"
+ },
+ {
+ "name": "HIP 115501",
+ "pos_x": -14.375,
+ "pos_y": -243,
+ "pos_z": 83.75,
+ "stations": "Zhu Station,Bolton Hub,Nomen Vision,Piserchia Horizons,Cabot Bastion"
+ },
+ {
+ "name": "HIP 115505",
+ "pos_x": -55.09375,
+ "pos_y": -168.6875,
+ "pos_z": 39.09375,
+ "stations": "Xuesen Station,de Kamp Hub,Molchanov Station,Obruchev Installation"
+ },
+ {
+ "name": "HIP 115581",
+ "pos_x": 86.34375,
+ "pos_y": -194.96875,
+ "pos_z": 107.84375,
+ "stations": "Allen Reformatory"
+ },
+ {
+ "name": "HIP 115584",
+ "pos_x": -5.5625,
+ "pos_y": -179.3125,
+ "pos_z": 63.1875,
+ "stations": "Matthaus Olbers Relay,Brunner Landing,Rodrigues Installation"
+ },
+ {
+ "name": "HIP 115610",
+ "pos_x": -112.03125,
+ "pos_y": -54.3125,
+ "pos_z": -27.8125,
+ "stations": "Clement Colony,Baydukov Dock,Piccard Arsenal"
+ },
+ {
+ "name": "HIP 115655",
+ "pos_x": -170.0625,
+ "pos_y": -47.34375,
+ "pos_z": -55.375,
+ "stations": "Thurston Barracks"
+ },
+ {
+ "name": "HIP 115664",
+ "pos_x": -170.5,
+ "pos_y": -100.71875,
+ "pos_z": -36.4375,
+ "stations": "Grigson Plant,McQuay Beacon,Anthony Escape"
+ },
+ {
+ "name": "HIP 115697",
+ "pos_x": -109.375,
+ "pos_y": -124.875,
+ "pos_z": -2.0625,
+ "stations": "Hamilton Asylum"
+ },
+ {
+ "name": "HIP 115736",
+ "pos_x": 105.40625,
+ "pos_y": -237.15625,
+ "pos_z": 129.78125,
+ "stations": "Christy Base,Bass Base"
+ },
+ {
+ "name": "HIP 11575",
+ "pos_x": 116.84375,
+ "pos_y": -257.6875,
+ "pos_z": -30.78125,
+ "stations": "Daqing Platform,Molyneux Dock"
+ },
+ {
+ "name": "HIP 11585",
+ "pos_x": 144.09375,
+ "pos_y": -254.25,
+ "pos_z": -1.53125,
+ "stations": "Herschel Point,Hiraga Hub,Gunter Dock"
+ },
+ {
+ "name": "HIP 11586",
+ "pos_x": 27.28125,
+ "pos_y": -159.75,
+ "pos_z": -64.5625,
+ "stations": "Sitterly Terminal,Bingzhen Survey,Aldiss Survey,Vess' Inheritance"
+ },
+ {
+ "name": "HIP 115888",
+ "pos_x": -2.15625,
+ "pos_y": -182.875,
+ "pos_z": 62.78125,
+ "stations": "Pilyugin Landing"
+ },
+ {
+ "name": "HIP 115917",
+ "pos_x": 45.21875,
+ "pos_y": -170.875,
+ "pos_z": 78.9375,
+ "stations": "Vlaicu Terminal,van den Hove Gateway"
+ },
+ {
+ "name": "HIP 115929",
+ "pos_x": 50.59375,
+ "pos_y": -183.375,
+ "pos_z": 85.5,
+ "stations": "Bahcall Hub,Kirby City,Wnuk-Lipinski Settlement,Fu Settlement,Gordon Landing,Collins Vista,Hill Horizons,Goodricke Dock,Giacobini Base,Bailly Dock,Hickman Terminal,Poyser Ring,Addams Orbital,Anderson City,Struve Vision"
+ },
+ {
+ "name": "HIP 115937",
+ "pos_x": 24.21875,
+ "pos_y": -148.71875,
+ "pos_z": 61.96875,
+ "stations": "Wheeler's Exile"
+ },
+ {
+ "name": "HIP 115951",
+ "pos_x": -66.625,
+ "pos_y": -105.09375,
+ "pos_z": 7.21875,
+ "stations": "Bunsen Port,Somerset Holdings,Halsell Depot"
+ },
+ {
+ "name": "HIP 11598",
+ "pos_x": 123.75,
+ "pos_y": -127.59375,
+ "pos_z": 50.875,
+ "stations": "Coggia's Progress,van Rhijn Horizons,Emshwiller Hub"
+ },
+ {
+ "name": "HIP 11600",
+ "pos_x": 32.03125,
+ "pos_y": -150.46875,
+ "pos_z": -54.6875,
+ "stations": "Draper Orbital,Ferguson Port,Allen Orbital"
+ },
+ {
+ "name": "HIP 116033",
+ "pos_x": -26.625,
+ "pos_y": -131.1875,
+ "pos_z": 32.96875,
+ "stations": "Auwers Terminal,Turzillo Barracks,Davies Port,Arend Terminal"
+ },
+ {
+ "name": "HIP 116045",
+ "pos_x": 35.875,
+ "pos_y": -181.78125,
+ "pos_z": 77.5,
+ "stations": "Balog Prospect,Alfven Prospect,Lagrange Platform,Bruce Penal colony,Godel Vista"
+ },
+ {
+ "name": "HIP 116066",
+ "pos_x": 147.59375,
+ "pos_y": -174.8125,
+ "pos_z": 124.15625,
+ "stations": "Severin Dock,Jones Prospect,Brosnatch Landing"
+ },
+ {
+ "name": "HIP 116074",
+ "pos_x": -15.5625,
+ "pos_y": -195.28125,
+ "pos_z": 59.125,
+ "stations": "Haro Dock,Brunel Base,Islam Vision"
+ },
+ {
+ "name": "HIP 116122",
+ "pos_x": 45.125,
+ "pos_y": -140.25,
+ "pos_z": 67.03125,
+ "stations": "Bingzhen Plant"
+ },
+ {
+ "name": "HIP 116125",
+ "pos_x": -48.5,
+ "pos_y": -193.59375,
+ "pos_z": 43.5,
+ "stations": "Vess Dock,Petaja Silo,Carpenter Mines,Michell Dock"
+ },
+ {
+ "name": "HIP 116154",
+ "pos_x": 19.28125,
+ "pos_y": -149.21875,
+ "pos_z": 58.40625,
+ "stations": "Elst Platform,Vancouver Keep"
+ },
+ {
+ "name": "HIP 116213",
+ "pos_x": -96.9375,
+ "pos_y": -79.1875,
+ "pos_z": -16.875,
+ "stations": "Yolen Hub,Wiener Dock,Brackett Oasis"
+ },
+ {
+ "name": "HIP 116222",
+ "pos_x": -155.21875,
+ "pos_y": -40,
+ "pos_z": -55.8125,
+ "stations": "Adams Dock,Siemens Orbital,Bosch Platform"
+ },
+ {
+ "name": "HIP 116232",
+ "pos_x": 103.75,
+ "pos_y": -231.59375,
+ "pos_z": 122.71875,
+ "stations": "Westerhout's Claim,Wolszczan Base"
+ },
+ {
+ "name": "HIP 116263",
+ "pos_x": 91.59375,
+ "pos_y": -234.09375,
+ "pos_z": 117.78125,
+ "stations": "Maury Enterprise,Sheepshanks Colony,Delany Enterprise"
+ },
+ {
+ "name": "HIP 116298",
+ "pos_x": 37.78125,
+ "pos_y": -224.46875,
+ "pos_z": 90.28125,
+ "stations": "Roth Works,Seitter Terminal"
+ },
+ {
+ "name": "HIP 116334",
+ "pos_x": -88.4375,
+ "pos_y": -101.59375,
+ "pos_z": -6.71875,
+ "stations": "Russell Colony,Robinson Port,Garcia Prospect"
+ },
+ {
+ "name": "HIP 116351",
+ "pos_x": -136.34375,
+ "pos_y": -69.40625,
+ "pos_z": -38.8125,
+ "stations": "Cook Terminal,McQuay Installation,Brust Point"
+ },
+ {
+ "name": "HIP 116352",
+ "pos_x": -51.09375,
+ "pos_y": -160.8125,
+ "pos_z": 29.0625,
+ "stations": "Mattei Estate,Moore Refinery,McIntyre Camp"
+ },
+ {
+ "name": "HIP 116360",
+ "pos_x": -152.71875,
+ "pos_y": -66.28125,
+ "pos_z": -47.25,
+ "stations": "Kandel Colony,Maine Arsenal,Biggle Oasis,Slonczewski Base"
+ },
+ {
+ "name": "HIP 116377",
+ "pos_x": 38.84375,
+ "pos_y": -172.9375,
+ "pos_z": 73.1875,
+ "stations": "van Royen Hub,Abell Station,Wickramasinghe Terminal,Eddington Landing"
+ },
+ {
+ "name": "HIP 116402",
+ "pos_x": -85.25,
+ "pos_y": -152.46875,
+ "pos_z": 10.46875,
+ "stations": "Chapman Refinery"
+ },
+ {
+ "name": "HIP 116410",
+ "pos_x": -68.78125,
+ "pos_y": -100,
+ "pos_z": 0.96875,
+ "stations": "Dirac Point,Sellers Terminal,Kozeyev Terminal"
+ },
+ {
+ "name": "HIP 116436",
+ "pos_x": -16.75,
+ "pos_y": -120.59375,
+ "pos_z": 30.90625,
+ "stations": "Konscak Observatory,von Bellingshausen Base,Esclangon Station,Davies Orbital,Payne-Gaposchkin Station,Balmer Terminal"
+ },
+ {
+ "name": "HIP 116454",
+ "pos_x": -99.71875,
+ "pos_y": -154.0625,
+ "pos_z": 3.9375,
+ "stations": "Alexandrov Station,Silves Depot"
+ },
+ {
+ "name": "HIP 116460",
+ "pos_x": 22,
+ "pos_y": -122.25,
+ "pos_z": 48.84375,
+ "stations": "Chadwick Point,Mil Landing,Bruck Vision,Delaunay Prospect"
+ },
+ {
+ "name": "HIP 116482",
+ "pos_x": -30.59375,
+ "pos_y": -206.5,
+ "pos_z": 51.59375,
+ "stations": "Landis Landing,Wales Platform,Xuesen Terminal,Lavochkin Observatory"
+ },
+ {
+ "name": "HIP 116492",
+ "pos_x": -5.65625,
+ "pos_y": -131.625,
+ "pos_z": 39.0625,
+ "stations": "Williams Keep,Bode Port"
+ },
+ {
+ "name": "HIP 116500",
+ "pos_x": 59.96875,
+ "pos_y": -200.25,
+ "pos_z": 90.46875,
+ "stations": "Lyne Prospect,Balog Platform"
+ },
+ {
+ "name": "HIP 116510",
+ "pos_x": 37.21875,
+ "pos_y": -235.84375,
+ "pos_z": 91.28125,
+ "stations": "Boss Enterprise"
+ },
+ {
+ "name": "HIP 116519",
+ "pos_x": -49.90625,
+ "pos_y": -161.5625,
+ "pos_z": 28.09375,
+ "stations": "Flammarion Dock,Akers Installation"
+ },
+ {
+ "name": "HIP 116528",
+ "pos_x": 4.59375,
+ "pos_y": -254.46875,
+ "pos_z": 81.90625,
+ "stations": "Bouvard Colony"
+ },
+ {
+ "name": "HIP 116553",
+ "pos_x": 86.0625,
+ "pos_y": -229.78125,
+ "pos_z": 111.0625,
+ "stations": "Schwarzschild's Exile,Arp Port,Jael Installation"
+ },
+ {
+ "name": "HIP 116554",
+ "pos_x": 29.28125,
+ "pos_y": -173.15625,
+ "pos_z": 67.4375,
+ "stations": "d'Arrest Orbital,Dumont Settlement"
+ },
+ {
+ "name": "HIP 11661",
+ "pos_x": 105.25,
+ "pos_y": -177.75,
+ "pos_z": 2.53125,
+ "stations": "Naburimannu Market,Heaviside Survey,Hornoch Station,Payne's Folly,Okuni Landing,Metcalf Vista"
+ },
+ {
+ "name": "HIP 116612",
+ "pos_x": 86.375,
+ "pos_y": -205.6875,
+ "pos_z": 103.1875,
+ "stations": "Drake Landing,Reinhold Installation"
+ },
+ {
+ "name": "HIP 116616",
+ "pos_x": -145.96875,
+ "pos_y": -33.21875,
+ "pos_z": -56.625,
+ "stations": "Burnham Orbital,Lopez de Villalobos Port,Luiken Arsenal"
+ },
+ {
+ "name": "HIP 116647",
+ "pos_x": 138,
+ "pos_y": -173.1875,
+ "pos_z": 116.6875,
+ "stations": "Dubyago Port,Kotzebue Landing,Kuiper Colony"
+ },
+ {
+ "name": "HIP 116663",
+ "pos_x": 56.96875,
+ "pos_y": -262.59375,
+ "pos_z": 106.8125,
+ "stations": "Mies van der Rohe Port"
+ },
+ {
+ "name": "HIP 116710",
+ "pos_x": 73.71875,
+ "pos_y": -157.59375,
+ "pos_z": 81.96875,
+ "stations": "Anastase Perrotin Orbital,Chretien Oudemans Hub,Adams Terminal,Guth Vista,Phillips Vision,Hayashi Terminal,Frost Hub,Kaluta Hub,Pollas Orbital,Green Vision,Tavares Reach"
+ },
+ {
+ "name": "HIP 116807",
+ "pos_x": -28.5625,
+ "pos_y": -188.375,
+ "pos_z": 43.03125,
+ "stations": "Cayley Mines,Arzachel Orbital,Goldreich Orbital,Shaara Terminal"
+ },
+ {
+ "name": "HIP 116890",
+ "pos_x": 40.625,
+ "pos_y": -184.71875,
+ "pos_z": 73.3125,
+ "stations": "Rosenberger Prospect,Matheson Vista,Gulyaev Base,Rechtin Vision"
+ },
+ {
+ "name": "HIP 116905",
+ "pos_x": 14.25,
+ "pos_y": -112.75,
+ "pos_z": 39.6875,
+ "stations": "Roth Vision,Perga Survey,Lozino-Lozinskiy City"
+ },
+ {
+ "name": "HIP 116910",
+ "pos_x": 2.3125,
+ "pos_y": -199.09375,
+ "pos_z": 59.34375,
+ "stations": "Puiseux Terminal,Chertovsky's Folly,Trujillo Platform,Sei Hub,Bennett Base"
+ },
+ {
+ "name": "HIP 11693",
+ "pos_x": 136.875,
+ "pos_y": -186.71875,
+ "pos_z": 28.90625,
+ "stations": "Dawes Station,Sugano Terminal,Qushji Beacon,Quick Prospect,Bunch Mine"
+ },
+ {
+ "name": "HIP 116939",
+ "pos_x": 113.84375,
+ "pos_y": -98.25,
+ "pos_z": 82.03125,
+ "stations": "Flammarion Vision,Alfven Station"
+ },
+ {
+ "name": "HIP 116970",
+ "pos_x": 24.84375,
+ "pos_y": -244.375,
+ "pos_z": 82.375,
+ "stations": "Pannekoek Mines,Hickam Dock,Vaez de Torres Beacon"
+ },
+ {
+ "name": "HIP 116973",
+ "pos_x": -35.65625,
+ "pos_y": -141.65625,
+ "pos_z": 24.1875,
+ "stations": "Dyson Port,Frazetta Survey,Lopez de Villalobos Holdings"
+ },
+ {
+ "name": "HIP 116984",
+ "pos_x": -80.8125,
+ "pos_y": -130,
+ "pos_z": -0.625,
+ "stations": "Descartes Colony,Whitelaw Reach"
+ },
+ {
+ "name": "HIP 117002",
+ "pos_x": -58,
+ "pos_y": -151.84375,
+ "pos_z": 16.25,
+ "stations": "Detmer Landing,Parise Hangar,Volterra Enterprise,Warner Settlement"
+ },
+ {
+ "name": "HIP 117014",
+ "pos_x": 118.78125,
+ "pos_y": -159.84375,
+ "pos_z": 101.90625,
+ "stations": "Bruck Market,Schade Camp,Ponce de Leon Bastion"
+ },
+ {
+ "name": "HIP 117029",
+ "pos_x": -136.03125,
+ "pos_y": -8.96875,
+ "pos_z": -61.71875,
+ "stations": "Bella Port,Neumann Dock,Armstrong Orbital,Kippax Holdings,Martins Holdings"
+ },
+ {
+ "name": "HIP 117035",
+ "pos_x": 129.28125,
+ "pos_y": -151.96875,
+ "pos_z": 104.53125,
+ "stations": "Brackett Installation,Kapteyn Port,Mayer Platform,Pilcher Survey"
+ },
+ {
+ "name": "HIP 117036",
+ "pos_x": -26.40625,
+ "pos_y": -148.9375,
+ "pos_z": 30.0625,
+ "stations": "Wegener Station,Orbik Observatory,Zetford Depot"
+ },
+ {
+ "name": "HIP 117064",
+ "pos_x": -23.84375,
+ "pos_y": -206.90625,
+ "pos_z": 47.46875,
+ "stations": "Helffrich Dock,Sagan Ring,Molchanov Orbital,Hamilton Beacon"
+ },
+ {
+ "name": "HIP 117091",
+ "pos_x": -8.0625,
+ "pos_y": -181.59375,
+ "pos_z": 47.53125,
+ "stations": "Busch Point,Vakhmistrov Survey,De Orbital"
+ },
+ {
+ "name": "HIP 117114",
+ "pos_x": 125.375,
+ "pos_y": -242.8125,
+ "pos_z": 127.9375,
+ "stations": "Molyneux Horizons,Narlikar Vision,Abbot Enterprise,Gold Hub"
+ },
+ {
+ "name": "HIP 117121",
+ "pos_x": -36.96875,
+ "pos_y": -160.96875,
+ "pos_z": 27.625,
+ "stations": "Vlaicu Port,Fan Settlement"
+ },
+ {
+ "name": "HIP 117177",
+ "pos_x": -145,
+ "pos_y": -61.78125,
+ "pos_z": -52.125,
+ "stations": "Dias Holdings,Swift Holdings"
+ },
+ {
+ "name": "HIP 117187",
+ "pos_x": 45.34375,
+ "pos_y": -191.15625,
+ "pos_z": 74.625,
+ "stations": "Ashbrook Hub,Alexandria Hub,Lilienthal Survey,Hadamard Barracks,Walotsky Orbital,Herzog Dock,Adkins Dock,d'Allonville Lab"
+ },
+ {
+ "name": "HIP 117235",
+ "pos_x": -103.71875,
+ "pos_y": -122.375,
+ "pos_z": -16.25,
+ "stations": "Grzimek Enterprise,Crown Enterprise,Viete Laboratory"
+ },
+ {
+ "name": "HIP 117236",
+ "pos_x": -89.1875,
+ "pos_y": -106.84375,
+ "pos_z": -13.53125,
+ "stations": "Paulmier de Gonneville City"
+ },
+ {
+ "name": "HIP 117254",
+ "pos_x": 27.09375,
+ "pos_y": -240.8125,
+ "pos_z": 78.78125,
+ "stations": "Hirayama Port,Perga Hub"
+ },
+ {
+ "name": "HIP 117258",
+ "pos_x": -49.78125,
+ "pos_y": -115.34375,
+ "pos_z": 7.53125,
+ "stations": "Patsayev Port,Deluc Port,Gagnan Mines"
+ },
+ {
+ "name": "HIP 11728",
+ "pos_x": -49.9375,
+ "pos_y": -73.90625,
+ "pos_z": -94.46875,
+ "stations": "Ford Platform,Franklin Vista,Leeuwenhoek Relay"
+ },
+ {
+ "name": "HIP 117288",
+ "pos_x": -6.375,
+ "pos_y": -138.125,
+ "pos_z": 34.40625,
+ "stations": "Bennett Orbital,Havilland Beacon,Perlmutter City,Lalande Terminal,Stechkin Silo,Piano Terminal"
+ },
+ {
+ "name": "HIP 1173",
+ "pos_x": 29.5625,
+ "pos_y": -237.125,
+ "pos_z": 52.65625,
+ "stations": "Mies van der Rohe Prospect,Mayor Legacy"
+ },
+ {
+ "name": "HIP 117322",
+ "pos_x": 98.5625,
+ "pos_y": -244.625,
+ "pos_z": 113.5,
+ "stations": "Brundage Depot,Kreutz's Claim"
+ },
+ {
+ "name": "HIP 117336",
+ "pos_x": -92.25,
+ "pos_y": -85.34375,
+ "pos_z": -21.625,
+ "stations": "Piccard Settlement,Gardner Installation"
+ },
+ {
+ "name": "HIP 117391",
+ "pos_x": -52.25,
+ "pos_y": -178,
+ "pos_z": 22.03125,
+ "stations": "Molchanov Terminal,Luyten Orbital,Emshwiller Installation,Lowry Vision,Woodroffe Point"
+ },
+ {
+ "name": "HIP 117399",
+ "pos_x": -42.9375,
+ "pos_y": -169.1875,
+ "pos_z": 24.125,
+ "stations": "Weber Relay,Efremov Point"
+ },
+ {
+ "name": "HIP 117427",
+ "pos_x": -48.34375,
+ "pos_y": -152.15625,
+ "pos_z": 16.6875,
+ "stations": "Thomson Port,Jacobi Port,Fisk Relay"
+ },
+ {
+ "name": "HIP 117461",
+ "pos_x": -99.0625,
+ "pos_y": -119.71875,
+ "pos_z": -16.8125,
+ "stations": "Rushd Terminal,Kondakova Legacy"
+ },
+ {
+ "name": "HIP 117471",
+ "pos_x": 28.15625,
+ "pos_y": -231.25,
+ "pos_z": 74.25,
+ "stations": "Pilcher Enterprise,Takamizawa Orbital,Giles Landing"
+ },
+ {
+ "name": "HIP 117493",
+ "pos_x": 99.625,
+ "pos_y": -160.84375,
+ "pos_z": 90.53125,
+ "stations": "Peltier Survey,Gould Vision"
+ },
+ {
+ "name": "HIP 117499",
+ "pos_x": 107.6875,
+ "pos_y": -162.0625,
+ "pos_z": 94.75,
+ "stations": "Luther Vision,Vakhmistrov Settlement"
+ },
+ {
+ "name": "HIP 117627",
+ "pos_x": 5.28125,
+ "pos_y": -196.59375,
+ "pos_z": 52.28125,
+ "stations": "Aller Station,MacDonald Bastion"
+ },
+ {
+ "name": "HIP 117668",
+ "pos_x": -3.1875,
+ "pos_y": -222.84375,
+ "pos_z": 54.28125,
+ "stations": "Marius Colony"
+ },
+ {
+ "name": "HIP 117706",
+ "pos_x": 29.90625,
+ "pos_y": -150.125,
+ "pos_z": 52.125,
+ "stations": "Carpenter Port,Mrkos Keep"
+ },
+ {
+ "name": "HIP 117783",
+ "pos_x": 107.46875,
+ "pos_y": -170,
+ "pos_z": 95.15625,
+ "stations": "Lane City"
+ },
+ {
+ "name": "HIP 117831",
+ "pos_x": 47.125,
+ "pos_y": -256.78125,
+ "pos_z": 85.6875,
+ "stations": "Peters Station,Tuttle Relay,Auld Legacy"
+ },
+ {
+ "name": "HIP 117846",
+ "pos_x": -105,
+ "pos_y": -101.78125,
+ "pos_z": -28.03125,
+ "stations": "Manakov Hub,Sinclair Depot,Nakaya's Folly"
+ },
+ {
+ "name": "HIP 117865",
+ "pos_x": -6.25,
+ "pos_y": -139.84375,
+ "pos_z": 30.375,
+ "stations": "Rechtin Gateway,Bothezat Hub,Fox Terminal,McKean Orbital,Giles Holdings,Sy Holdings,Lee Station,Roskam Hub,Beg Hub,Soper Installation,Schumacher Port,Gessi Works,Nomen Gateway"
+ },
+ {
+ "name": "HIP 117870",
+ "pos_x": -138.84375,
+ "pos_y": -90.03125,
+ "pos_z": -48.09375,
+ "stations": "Feustel Camp,Conway Survey"
+ },
+ {
+ "name": "HIP 117878",
+ "pos_x": 122.375,
+ "pos_y": -254.1875,
+ "pos_z": 122.09375,
+ "stations": "Velho Horizons,Uto Arsenal"
+ },
+ {
+ "name": "HIP 117883",
+ "pos_x": 14.09375,
+ "pos_y": -242.5625,
+ "pos_z": 64.9375,
+ "stations": "Aller Hub,Pogson Dock,Cook Horizons"
+ },
+ {
+ "name": "HIP 117895",
+ "pos_x": 37.53125,
+ "pos_y": -161.53125,
+ "pos_z": 57.28125,
+ "stations": "McMullen Holdings,Carnera Hub,Wasden Hub"
+ },
+ {
+ "name": "HIP 117903",
+ "pos_x": 33.09375,
+ "pos_y": -252.1875,
+ "pos_z": 76.53125,
+ "stations": "Asami Camp,Reichelt Depot"
+ },
+ {
+ "name": "HIP 117911",
+ "pos_x": -55.8125,
+ "pos_y": -163.34375,
+ "pos_z": 10.65625,
+ "stations": "Wallerstein Vision,Niemeyer Terminal,Ings Relay"
+ },
+ {
+ "name": "HIP 117958",
+ "pos_x": -27.8125,
+ "pos_y": -133.75,
+ "pos_z": 17.34375,
+ "stations": "Esposito Settlement,Beekman Market"
+ },
+ {
+ "name": "HIP 11796",
+ "pos_x": -115.5625,
+ "pos_y": -12.6875,
+ "pos_z": -125.34375,
+ "stations": "Card's Folly"
+ },
+ {
+ "name": "HIP 117960",
+ "pos_x": -75.1875,
+ "pos_y": -126.4375,
+ "pos_z": -8.28125,
+ "stations": "Taylor Port,Crown Ring"
+ },
+ {
+ "name": "HIP 117965",
+ "pos_x": -2.4375,
+ "pos_y": -193.65625,
+ "pos_z": 44.15625,
+ "stations": "Mooz Dock,Fearn Horizons,Korolev Colony,Carr Base"
+ },
+ {
+ "name": "HIP 117972",
+ "pos_x": -126.59375,
+ "pos_y": -96.4375,
+ "pos_z": -41.3125,
+ "stations": "Flynn Terminal,Sauma Port,Lukyanenko Terminal,Fontana Installation"
+ },
+ {
+ "name": "HIP 117975",
+ "pos_x": -8.5,
+ "pos_y": -186.6875,
+ "pos_z": 39.375,
+ "stations": "Dyr Hub,Dias' Progress,Ohain Vision,Shea Hub,Leuschner Gateway,Benyovszky's Folly,Tarter Port,Davis Survey,Dashiell Landing"
+ },
+ {
+ "name": "HIP 118020",
+ "pos_x": -62.75,
+ "pos_y": -157.4375,
+ "pos_z": 4.625,
+ "stations": "Oshima Dock,Drake Landing,Houtman Survey"
+ },
+ {
+ "name": "HIP 118040",
+ "pos_x": 43.40625,
+ "pos_y": -154.96875,
+ "pos_z": 57.6875,
+ "stations": "Flagg Bastion,Mechain Mines"
+ },
+ {
+ "name": "HIP 118045",
+ "pos_x": 43.28125,
+ "pos_y": -240.21875,
+ "pos_z": 77.21875,
+ "stations": "Anastase Perrotin Prospect"
+ },
+ {
+ "name": "HIP 118061",
+ "pos_x": 98.625,
+ "pos_y": -177.625,
+ "pos_z": 90.71875,
+ "stations": "Westphal Station,Youll Depot,Kepler Settlement"
+ },
+ {
+ "name": "HIP 118062",
+ "pos_x": 102.21875,
+ "pos_y": -154.9375,
+ "pos_z": 87.34375,
+ "stations": "Allen Settlement,Arago Prospect"
+ },
+ {
+ "name": "HIP 118079",
+ "pos_x": 150.15625,
+ "pos_y": -174.75,
+ "pos_z": 116.1875,
+ "stations": "Encke Port"
+ },
+ {
+ "name": "HIP 118091",
+ "pos_x": 34.71875,
+ "pos_y": -169.09375,
+ "pos_z": 56.09375,
+ "stations": "Jacobi Installation,Gurshtein Landing,Pickering Platform,Baillaud Colony"
+ },
+ {
+ "name": "HIP 118106",
+ "pos_x": 99.59375,
+ "pos_y": -219.5,
+ "pos_z": 100.46875,
+ "stations": "Honda Platform,Kuchner Depot,Mieville Beacon"
+ },
+ {
+ "name": "HIP 118115",
+ "pos_x": -57.25,
+ "pos_y": -145.625,
+ "pos_z": 3.78125,
+ "stations": "Hartsfield Orbital,Jahn Works,Leoniceno Keep"
+ },
+ {
+ "name": "HIP 118127",
+ "pos_x": -1.9375,
+ "pos_y": -216.65625,
+ "pos_z": 47.9375,
+ "stations": "Keldysh Station,Palisa Arsenal,Roddenberry Enterprise"
+ },
+ {
+ "name": "HIP 118146",
+ "pos_x": -61.40625,
+ "pos_y": -140.21875,
+ "pos_z": 0.15625,
+ "stations": "Maxwell Hangar,Northrop Dock"
+ },
+ {
+ "name": "HIP 118169",
+ "pos_x": 80.625,
+ "pos_y": -193.375,
+ "pos_z": 84.40625,
+ "stations": "Peters Market,Carnera Terminal,Brahmagupta Gateway,Reber Port,Montanari Hub,Erdos Arsenal,Tanaka Survey,Parkinson Base"
+ },
+ {
+ "name": "HIP 11819",
+ "pos_x": 148.15625,
+ "pos_y": -159.4375,
+ "pos_z": 55.5,
+ "stations": "Bingzhen's Claim"
+ },
+ {
+ "name": "HIP 118210",
+ "pos_x": 16.875,
+ "pos_y": -227.53125,
+ "pos_z": 59,
+ "stations": "Furukawa Hub,Hirn Station,Thurston Penal colony,Fife Installation"
+ },
+ {
+ "name": "HIP 118213",
+ "pos_x": -131.71875,
+ "pos_y": -79.59375,
+ "pos_z": -49.9375,
+ "stations": "Lorenz Hub,Papin Port,Resnick Bastion,Herbert Survey"
+ },
+ {
+ "name": "HIP 118228",
+ "pos_x": 106.34375,
+ "pos_y": -207.25,
+ "pos_z": 100.28125,
+ "stations": "Payne-Scott Dock,Nakamura Escape,Young Prospect"
+ },
+ {
+ "name": "HIP 118247",
+ "pos_x": -56.6875,
+ "pos_y": -162.03125,
+ "pos_z": 6.53125,
+ "stations": "Jekhowsky Horizons,Hodgson Vision"
+ },
+ {
+ "name": "HIP 118251",
+ "pos_x": -118,
+ "pos_y": -47.84375,
+ "pos_z": -50.125,
+ "stations": "Voss Dock,Kizim Hub,Hennen Dock,Feynman Terminal"
+ },
+ {
+ "name": "HIP 118311",
+ "pos_x": 9.40625,
+ "pos_y": -123.5,
+ "pos_z": 31.59375,
+ "stations": "Lubbock Market,Jewitt Terminal,Winthrop Prospect,Janifer Prospect"
+ },
+ {
+ "name": "HIP 118321",
+ "pos_x": 79.3125,
+ "pos_y": -136.8125,
+ "pos_z": 70.46875,
+ "stations": "Herzog Gateway,Herschel Vision,Glass Silo,De Lay Vision,McQuarrie City"
+ },
+ {
+ "name": "HIP 11847",
+ "pos_x": -111.375,
+ "pos_y": -73.625,
+ "pos_z": -158.34375,
+ "stations": "Bombelli Colony,Rochon Vision,Cremona Base"
+ },
+ {
+ "name": "HIP 1185",
+ "pos_x": -77.1875,
+ "pos_y": -279.46875,
+ "pos_z": -0.3125,
+ "stations": "Mike's Rock"
+ },
+ {
+ "name": "HIP 11859",
+ "pos_x": 94.625,
+ "pos_y": -221.375,
+ "pos_z": -37.0625,
+ "stations": "Fedden Settlement"
+ },
+ {
+ "name": "HIP 1187",
+ "pos_x": -11,
+ "pos_y": -248.84375,
+ "pos_z": 31.75,
+ "stations": "Veron's Claim"
+ },
+ {
+ "name": "HIP 11886",
+ "pos_x": 185.4375,
+ "pos_y": -170.75,
+ "pos_z": 86.40625,
+ "stations": "Ore Installation,Fuchs Horizons"
+ },
+ {
+ "name": "HIP 11909",
+ "pos_x": 132.84375,
+ "pos_y": -133.40625,
+ "pos_z": 54.96875,
+ "stations": "Martiniere Ring,Schiltberger Relay,Froud Survey,Strzelecki Arena"
+ },
+ {
+ "name": "HIP 11913",
+ "pos_x": 166.25,
+ "pos_y": -252.9375,
+ "pos_z": 16.34375,
+ "stations": "Cherry Mines,Green Penal colony"
+ },
+ {
+ "name": "HIP 11917",
+ "pos_x": 60.59375,
+ "pos_y": -199.25,
+ "pos_z": -59.5,
+ "stations": "Tedin Holdings,Rasch Base,Asclepi Depot"
+ },
+ {
+ "name": "HIP 11923",
+ "pos_x": -103.28125,
+ "pos_y": -27.21875,
+ "pos_z": -122.71875,
+ "stations": "Matteucci Orbital,Usachov Station,Hermite Horizons"
+ },
+ {
+ "name": "HIP 11928",
+ "pos_x": 73.34375,
+ "pos_y": -219.3125,
+ "pos_z": -58.78125,
+ "stations": "Froud Camp,He Bastion"
+ },
+ {
+ "name": "HIP 11954",
+ "pos_x": 43.75,
+ "pos_y": -125.9375,
+ "pos_z": -32.28125,
+ "stations": "Banno Orbital,Bridger Horizons"
+ },
+ {
+ "name": "HIP 11958",
+ "pos_x": 85.25,
+ "pos_y": -209.8125,
+ "pos_z": -41.1875,
+ "stations": "Maunder Terminal,Roth Prospect,Schoening Vision,Menezes Horizons"
+ },
+ {
+ "name": "HIP 12005",
+ "pos_x": 21.1875,
+ "pos_y": -134.4375,
+ "pos_z": -61.21875,
+ "stations": "Arai Mine"
+ },
+ {
+ "name": "HIP 12018",
+ "pos_x": 162.9375,
+ "pos_y": -126.8125,
+ "pos_z": 89.625,
+ "stations": "Gora Terminal,Tan Holdings,Fowler Dock,Kent's Inheritance"
+ },
+ {
+ "name": "HIP 12040",
+ "pos_x": -113.6875,
+ "pos_y": -24.59375,
+ "pos_z": -132.78125,
+ "stations": "Brunton Hub,Heng Prospect"
+ },
+ {
+ "name": "HIP 12065",
+ "pos_x": -4.9375,
+ "pos_y": -106.09375,
+ "pos_z": -71.40625,
+ "stations": "Eisele Bastion,Jakes Dock"
+ },
+ {
+ "name": "HIP 12067",
+ "pos_x": -107.28125,
+ "pos_y": 2.96875,
+ "pos_z": -109.25,
+ "stations": "Vaucanson Gateway,Pinto Palace,Hunziker Terminal,Sullivan Market,Karlsefni Bastion"
+ },
+ {
+ "name": "HIP 12087",
+ "pos_x": -28.0625,
+ "pos_y": -111.40625,
+ "pos_z": -98.875,
+ "stations": "Mohun Terminal,Gaspar de Portola Escape"
+ },
+ {
+ "name": "HIP 12102",
+ "pos_x": 26.90625,
+ "pos_y": -184.65625,
+ "pos_z": -88,
+ "stations": "Cannon Relay"
+ },
+ {
+ "name": "HIP 12119",
+ "pos_x": 35.90625,
+ "pos_y": -124.28125,
+ "pos_z": -40.9375,
+ "stations": "Polikarpov Platform,Turner Terminal"
+ },
+ {
+ "name": "HIP 12155",
+ "pos_x": -40.25,
+ "pos_y": -107.59375,
+ "pos_z": -110,
+ "stations": "Chaviano Dock,Swanwick Installation,Sargent Survey"
+ },
+ {
+ "name": "HIP 12167",
+ "pos_x": 44.40625,
+ "pos_y": -178.53125,
+ "pos_z": -67.03125,
+ "stations": "Tikhonravov Port,Cleve's Folly,Cardano's Folly,Schommer's Claim"
+ },
+ {
+ "name": "HIP 12248",
+ "pos_x": 112.6875,
+ "pos_y": -224.8125,
+ "pos_z": -26.40625,
+ "stations": "Carpenter Settlement,Tietjen Depot"
+ },
+ {
+ "name": "HIP 12314",
+ "pos_x": -98.3125,
+ "pos_y": -77.4375,
+ "pos_z": -153.53125,
+ "stations": "Bentham Station,Blair Station,Mieville Landing,Coney Plant,Bernoulli Hub"
+ },
+ {
+ "name": "HIP 12361",
+ "pos_x": 103.5625,
+ "pos_y": -161.59375,
+ "pos_z": 3.46875,
+ "stations": "Comper Ring,Vess Gateway,Nelder Depot,Gamow Terminal"
+ },
+ {
+ "name": "HIP 12368",
+ "pos_x": 90.8125,
+ "pos_y": -186.21875,
+ "pos_z": -26.15625,
+ "stations": "Perrine Colony"
+ },
+ {
+ "name": "HIP 12424",
+ "pos_x": 55.3125,
+ "pos_y": -226.21875,
+ "pos_z": -90.8125,
+ "stations": "Nijland Hub,Mawson Depot"
+ },
+ {
+ "name": "HIP 12425",
+ "pos_x": 46.8125,
+ "pos_y": -185.84375,
+ "pos_z": -73.1875,
+ "stations": "Allen St. John Colony,Sorayama Prospect,Sabine's Folly,McDevitt Lab"
+ },
+ {
+ "name": "HIP 12480",
+ "pos_x": 38.03125,
+ "pos_y": -176.625,
+ "pos_z": -77.25,
+ "stations": "Gentil Platform,Pogson Platform,Gibson Hub"
+ },
+ {
+ "name": "HIP 12509",
+ "pos_x": -86.9375,
+ "pos_y": -51.71875,
+ "pos_z": -127,
+ "stations": "Ashton Orbital,Ashton Prospect,Hovgaard Beacon,Carlisle Depot"
+ },
+ {
+ "name": "HIP 12581",
+ "pos_x": 75.59375,
+ "pos_y": -195.6875,
+ "pos_z": -51.5625,
+ "stations": "Eggleton Beacon"
+ },
+ {
+ "name": "HIP 12590",
+ "pos_x": 77.96875,
+ "pos_y": -173.6875,
+ "pos_z": -34.3125,
+ "stations": "Flammarion Mines,Uto Mines"
+ },
+ {
+ "name": "HIP 12605",
+ "pos_x": 44.9375,
+ "pos_y": -129.625,
+ "pos_z": -39.90625,
+ "stations": "Rigaux Gateway,Watt-Evans Relay,Agrippa Orbital,Havilland Gateway"
+ },
+ {
+ "name": "HIP 12635",
+ "pos_x": -86.90625,
+ "pos_y": -53.1875,
+ "pos_z": -129.15625,
+ "stations": "Gelfand Orbital,Rasch Orbital,Kiernan Ring,Covey Beacon,Burgess Camp"
+ },
+ {
+ "name": "HIP 12638",
+ "pos_x": -78.3125,
+ "pos_y": -47.9375,
+ "pos_z": -116.4375,
+ "stations": "Galouye Gateway,Guerrero Hub,Stuart Orbital,Allen Forum"
+ },
+ {
+ "name": "HIP 12716",
+ "pos_x": 52.53125,
+ "pos_y": -119.125,
+ "pos_z": -25.625,
+ "stations": "Bond Port,Terry Station,Le Guin Vision,Fidalgo Enterprise,Bachman Enterprise,Evangelisti Dock,Miklouho-Maclay Silo,Bruce Enterprise,Muramatsu Camp,Milnor Horizons"
+ },
+ {
+ "name": "HIP 12764",
+ "pos_x": 0.25,
+ "pos_y": -112.59375,
+ "pos_z": -77.9375,
+ "stations": "Lloyd Settlement,Johnson Prospect"
+ },
+ {
+ "name": "HIP 12766",
+ "pos_x": 120.21875,
+ "pos_y": -130.03125,
+ "pos_z": 39.3125,
+ "stations": "Regiomontanus Holdings,Alden Vision,Hoffleit Arsenal,Thesiger Terminal"
+ },
+ {
+ "name": "HIP 12779",
+ "pos_x": 35.96875,
+ "pos_y": -128.46875,
+ "pos_z": -50.65625,
+ "stations": "Rechtin Vision,Napier Beacon,Naubakht Terminal,McMullen Penal colony,Parker Dock"
+ },
+ {
+ "name": "HIP 12785",
+ "pos_x": 163.375,
+ "pos_y": -207.875,
+ "pos_z": 31.53125,
+ "stations": "Langley Depot,Clark Prospect"
+ },
+ {
+ "name": "HIP 12804",
+ "pos_x": -117.15625,
+ "pos_y": -38.65625,
+ "pos_z": -153.71875,
+ "stations": "Alvarado Holdings"
+ },
+ {
+ "name": "HIP 12837",
+ "pos_x": 28.84375,
+ "pos_y": -121.03125,
+ "pos_z": -53.84375,
+ "stations": "Celebi Refinery"
+ },
+ {
+ "name": "HIP 12860",
+ "pos_x": 42.84375,
+ "pos_y": -184.09375,
+ "pos_z": -83.34375,
+ "stations": "Young Relay,Marques Prospect,Gehry Platform"
+ },
+ {
+ "name": "HIP 12868",
+ "pos_x": 108.4375,
+ "pos_y": -218.375,
+ "pos_z": -36.5625,
+ "stations": "Boscovich Holdings,Meech Oasis,Langley Installation,Gessi Horizons"
+ },
+ {
+ "name": "HIP 12897",
+ "pos_x": 25.625,
+ "pos_y": -156.9375,
+ "pos_z": -83.5625,
+ "stations": "Osterbrock Prospect,Robinson's Inheritance"
+ },
+ {
+ "name": "HIP 1290",
+ "pos_x": -15.5,
+ "pos_y": -257.1875,
+ "pos_z": 28.96875,
+ "stations": "Harvey-Smith Platform,Altshuller Holdings"
+ },
+ {
+ "name": "HIP 12907",
+ "pos_x": 76.9375,
+ "pos_y": -219.03125,
+ "pos_z": -72,
+ "stations": "Struzan Colony,Pavlou Survey,Dubyago Survey"
+ },
+ {
+ "name": "HIP 12921",
+ "pos_x": 152.84375,
+ "pos_y": -111.4375,
+ "pos_z": 87.15625,
+ "stations": "Axon Dock,Ayers Vision,Hirn Port"
+ },
+ {
+ "name": "HIP 12954",
+ "pos_x": 70.5625,
+ "pos_y": -185.5,
+ "pos_z": -55.90625,
+ "stations": "Grushin Prospect,Andersson Landing,Trimble Hub"
+ },
+ {
+ "name": "HIP 12965",
+ "pos_x": 113.9375,
+ "pos_y": -231.28125,
+ "pos_z": -41.5625,
+ "stations": "Westerhout Holdings,Gaensler Vision,Whipple's Claim"
+ },
+ {
+ "name": "HIP 12966",
+ "pos_x": 1.5,
+ "pos_y": -160.5,
+ "pos_z": -113.75,
+ "stations": "Scalzi Prospect,Hausdorff Retreat,Rubruck Platform"
+ },
+ {
+ "name": "HIP 1299",
+ "pos_x": 6.59375,
+ "pos_y": -238.4375,
+ "pos_z": 38.4375,
+ "stations": "Baracchi Platform,Sargent Base"
+ },
+ {
+ "name": "HIP 1306",
+ "pos_x": -34.5,
+ "pos_y": -246.8125,
+ "pos_z": 16.59375,
+ "stations": "Burnham Mines,Pennington Mines"
+ },
+ {
+ "name": "HIP 13112",
+ "pos_x": 74.4375,
+ "pos_y": -164.9375,
+ "pos_z": -39.09375,
+ "stations": "Lubbock Vision,West Silo"
+ },
+ {
+ "name": "HIP 13129",
+ "pos_x": 120.1875,
+ "pos_y": -209.5,
+ "pos_z": -21.65625,
+ "stations": "Carsono Terminal,Pytheas Port,Maury Horizons,Kelleam Relay,Heinemann Hub,Bliss Orbital"
+ },
+ {
+ "name": "HIP 13159",
+ "pos_x": 133.96875,
+ "pos_y": -228.625,
+ "pos_z": -21,
+ "stations": "Fedden Relay"
+ },
+ {
+ "name": "HIP 13173",
+ "pos_x": -30.625,
+ "pos_y": -131.875,
+ "pos_z": -131.875,
+ "stations": "Ryman Orbital,Tavernier Dock,Langsdorff Relay,Dummer Hub"
+ },
+ {
+ "name": "HIP 13179",
+ "pos_x": -92.28125,
+ "pos_y": 30.875,
+ "pos_z": -79.34375,
+ "stations": "Noakes Dock,Avdeyev City,Shepard Base,Tuan Station,Huygens Settlement,Grigson Works"
+ },
+ {
+ "name": "HIP 13257",
+ "pos_x": -89.65625,
+ "pos_y": -60.96875,
+ "pos_z": -145.84375,
+ "stations": "Garnier City,Jordan Vision,Land Bastion"
+ },
+ {
+ "name": "HIP 13269",
+ "pos_x": -56.71875,
+ "pos_y": -108.8125,
+ "pos_z": -145.3125,
+ "stations": "Bond Station,Pournelle Dock,Gaultier de Varennes' Folly"
+ },
+ {
+ "name": "HIP 13285",
+ "pos_x": 71.9375,
+ "pos_y": -205.8125,
+ "pos_z": -75.375,
+ "stations": "Comper Point,Vuia Depot"
+ },
+ {
+ "name": "HIP 13291",
+ "pos_x": -66,
+ "pos_y": -66.71875,
+ "pos_z": -124.25,
+ "stations": "Godel Enterprise,Bordage Enterprise,Speke's Progress,Morgan Dock"
+ },
+ {
+ "name": "HIP 13317",
+ "pos_x": 78.5625,
+ "pos_y": -212.53125,
+ "pos_z": -73.65625,
+ "stations": "Tisserand Hub,Shimizu Survey,Wickramasinghe Gateway,Jeschke Holdings,Williams Vision"
+ },
+ {
+ "name": "HIP 1334",
+ "pos_x": -101.78125,
+ "pos_y": -96.5,
+ "pos_z": -43.15625,
+ "stations": "Higginbotham Survey"
+ },
+ {
+ "name": "HIP 13341",
+ "pos_x": 143.25,
+ "pos_y": -212.90625,
+ "pos_z": -1.875,
+ "stations": "Maunder Landing,Keeler Depot"
+ },
+ {
+ "name": "HIP 13350",
+ "pos_x": 102.3125,
+ "pos_y": -155.71875,
+ "pos_z": -4.1875,
+ "stations": "Olahus Terminal,Glass Terminal"
+ },
+ {
+ "name": "HIP 13366",
+ "pos_x": -39.625,
+ "pos_y": -130.8125,
+ "pos_z": -144.65625,
+ "stations": "Menzies Landing,Pribylov Beacon"
+ },
+ {
+ "name": "HIP 13410",
+ "pos_x": -18.09375,
+ "pos_y": -102.875,
+ "pos_z": -99.8125,
+ "stations": "Makeev Base"
+ },
+ {
+ "name": "HIP 13438",
+ "pos_x": -82.8125,
+ "pos_y": -61.125,
+ "pos_z": -140.84375,
+ "stations": "Erikson Landing"
+ },
+ {
+ "name": "HIP 13498",
+ "pos_x": 113.125,
+ "pos_y": -199.96875,
+ "pos_z": -28.375,
+ "stations": "Sitterly City"
+ },
+ {
+ "name": "HIP 13514",
+ "pos_x": 144.09375,
+ "pos_y": -230.875,
+ "pos_z": -17.75,
+ "stations": "Spassky's Folly,Kidinnu Point,Struve Settlement"
+ },
+ {
+ "name": "HIP 13536",
+ "pos_x": 113.53125,
+ "pos_y": -155.09375,
+ "pos_z": 6.8125,
+ "stations": "Trimble Outpost"
+ },
+ {
+ "name": "HIP 13564",
+ "pos_x": 44.21875,
+ "pos_y": -170.15625,
+ "pos_z": -84.3125,
+ "stations": "van Royen Vision,O'Neill Station,Cixin Depot,Finney Holdings"
+ },
+ {
+ "name": "HIP 13569",
+ "pos_x": 89.46875,
+ "pos_y": -212.0625,
+ "pos_z": -66.125,
+ "stations": "Jones' Pride,Couper Dock,Takahashi Horizons"
+ },
+ {
+ "name": "HIP 13644",
+ "pos_x": -47.84375,
+ "pos_y": -55.34375,
+ "pos_z": -99.0625,
+ "stations": "Davis Gateway,Herndon Penal colony,Piccard Arena"
+ },
+ {
+ "name": "HIP 13653",
+ "pos_x": -60.46875,
+ "pos_y": -48,
+ "pos_z": -107.6875,
+ "stations": "Vinge Dock,Williams Beacon,Vinge Survey,Dantec Survey,Amis Terminal"
+ },
+ {
+ "name": "HIP 13674",
+ "pos_x": -21.5,
+ "pos_y": -102.40625,
+ "pos_z": -107.0625,
+ "stations": "Stott Port,Ray Gateway,Leavitt Vision,Niven Keep,Barjavel Base"
+ },
+ {
+ "name": "HIP 13681",
+ "pos_x": 18.125,
+ "pos_y": -134.03125,
+ "pos_z": -87.1875,
+ "stations": "Gardner Works,Snodgrass Colony,Buckell Terminal"
+ },
+ {
+ "name": "HIP 13685",
+ "pos_x": 101.4375,
+ "pos_y": -155.46875,
+ "pos_z": -8.9375,
+ "stations": "Al-Khujandi Port,Tedin Depot,Titius Barracks,Kahn Platform"
+ },
+ {
+ "name": "HIP 13725",
+ "pos_x": 85.1875,
+ "pos_y": -183.96875,
+ "pos_z": -51.1875,
+ "stations": "Johnson Mine"
+ },
+ {
+ "name": "HIP 13750",
+ "pos_x": -22.375,
+ "pos_y": -87.125,
+ "pos_z": -96.625,
+ "stations": "Whitson City,Brongniart Gateway,Artyukhin Prospect,Slayton Hub,Kessel's Folly"
+ },
+ {
+ "name": "HIP 13758",
+ "pos_x": -1.28125,
+ "pos_y": -138.875,
+ "pos_z": -114.53125,
+ "stations": "Hillary's Exile,Klein Point"
+ },
+ {
+ "name": "HIP 13773",
+ "pos_x": -9.5,
+ "pos_y": -111.6875,
+ "pos_z": -102.03125,
+ "stations": "Blish Landing,Hyecho Dock"
+ },
+ {
+ "name": "HIP 13841",
+ "pos_x": 54.09375,
+ "pos_y": -114.28125,
+ "pos_z": -31.53125,
+ "stations": "Chandra Platform,May Orbital"
+ },
+ {
+ "name": "HIP 13864",
+ "pos_x": 114.84375,
+ "pos_y": -224.96875,
+ "pos_z": -52.875,
+ "stations": "Struve Platform,Vuia Survey"
+ },
+ {
+ "name": "HIP 13875",
+ "pos_x": -60.71875,
+ "pos_y": -45.09375,
+ "pos_z": -108,
+ "stations": "Duckworth Settlement,Parmitano Relay"
+ },
+ {
+ "name": "HIP 1389",
+ "pos_x": -103.21875,
+ "pos_y": -104.75,
+ "pos_z": -43.25,
+ "stations": "Daniel Gateway,Nikitin Ring,Kingsbury Orbital,Artin Orbital,Cummings Legacy,Person Beacon,Stokes Gateway"
+ },
+ {
+ "name": "HIP 13955",
+ "pos_x": 57.65625,
+ "pos_y": -187.5,
+ "pos_z": -90.125,
+ "stations": "Bainbridge Prospect,Bretnor Bastion,Bradley Survey"
+ },
+ {
+ "name": "HIP 13975",
+ "pos_x": 147.5,
+ "pos_y": -187.4375,
+ "pos_z": 14.65625,
+ "stations": "Finlay-Freundlich Depot,Dyson Barracks"
+ },
+ {
+ "name": "HIP 13977",
+ "pos_x": -27.125,
+ "pos_y": -121.34375,
+ "pos_z": -134.0625,
+ "stations": "Watts Landing"
+ },
+ {
+ "name": "HIP 13979",
+ "pos_x": 136.5625,
+ "pos_y": -208.90625,
+ "pos_z": -16.3125,
+ "stations": "Chretien Oudemans Station,Arzachel Vision,Ashman Orbital"
+ },
+ {
+ "name": "HIP 14001",
+ "pos_x": 12.5625,
+ "pos_y": -115.25,
+ "pos_z": -82.75,
+ "stations": "Sutcliffe Survey,Auer's Inheritance"
+ },
+ {
+ "name": "HIP 14007",
+ "pos_x": 64.78125,
+ "pos_y": -131.53125,
+ "pos_z": -35.3125,
+ "stations": "Foerster Bastion,Lozino-Lozinskiy Colony"
+ },
+ {
+ "name": "HIP 14031",
+ "pos_x": 74.21875,
+ "pos_y": -156.46875,
+ "pos_z": -45.8125,
+ "stations": "Porco City,Lem Settlement,Boss City,Boucher Vision,Lagrange Ring"
+ },
+ {
+ "name": "HIP 14045",
+ "pos_x": 139.96875,
+ "pos_y": -211.65625,
+ "pos_z": -15.6875,
+ "stations": "Dahm Hub,Morrill Gateway"
+ },
+ {
+ "name": "HIP 14106",
+ "pos_x": 130.28125,
+ "pos_y": -205.59375,
+ "pos_z": -23.1875,
+ "stations": "Kondo Port,Vonnegut Installation,Korolev Prospect,Kludze Holdings"
+ },
+ {
+ "name": "HIP 14116",
+ "pos_x": 126.53125,
+ "pos_y": -214.21875,
+ "pos_z": -35.28125,
+ "stations": "Brorsen Platform"
+ },
+ {
+ "name": "HIP 14134",
+ "pos_x": -79.5,
+ "pos_y": -39.34375,
+ "pos_z": -128.25,
+ "stations": "Thuot Horizons"
+ },
+ {
+ "name": "HIP 14157",
+ "pos_x": 28.75,
+ "pos_y": -137.9375,
+ "pos_z": -85.71875,
+ "stations": "Kagawa Platform,Chertovsky Hub"
+ },
+ {
+ "name": "HIP 14181",
+ "pos_x": -88.09375,
+ "pos_y": -26.25,
+ "pos_z": -127.5625,
+ "stations": "Bloomfield's Claim,Cormack Platform,Julian Refinery"
+ },
+ {
+ "name": "HIP 14182",
+ "pos_x": -81.375,
+ "pos_y": -12.125,
+ "pos_z": -107.28125,
+ "stations": "Fearn Bastion,Riemann Prospect,Bailey Depot"
+ },
+ {
+ "name": "HIP 1419",
+ "pos_x": 132.75,
+ "pos_y": -206,
+ "pos_z": 103.5,
+ "stations": "Dawes Port,Horrocks Dock,Westerhout Port,Zebrowski Port,Ziemkiewicz's Inheritance"
+ },
+ {
+ "name": "HIP 14194",
+ "pos_x": 1.03125,
+ "pos_y": -131.625,
+ "pos_z": -113.78125,
+ "stations": "Cartier Landing"
+ },
+ {
+ "name": "HIP 14211",
+ "pos_x": -41.59375,
+ "pos_y": -69.96875,
+ "pos_z": -110.78125,
+ "stations": "Williams Dock"
+ },
+ {
+ "name": "HIP 14249",
+ "pos_x": 48.21875,
+ "pos_y": -162.71875,
+ "pos_z": -85.875,
+ "stations": "Shen Platform,Bruce Landing,Timofeyevich Landing,Sugie Prospect,Honda Orbital,Clifford Holdings"
+ },
+ {
+ "name": "HIP 14253",
+ "pos_x": 75.875,
+ "pos_y": -206.8125,
+ "pos_z": -91.71875,
+ "stations": "Kirshner Dock,Adams Landing,Nesvadba Enterprise"
+ },
+ {
+ "name": "HIP 14257",
+ "pos_x": 131.0625,
+ "pos_y": -188.59375,
+ "pos_z": -9.78125,
+ "stations": "Weizsacker Gateway,Vavrova Hub,Hussey Mines,Antoniadi Point"
+ },
+ {
+ "name": "HIP 1427",
+ "pos_x": -94.625,
+ "pos_y": -104.5,
+ "pos_z": -38.78125,
+ "stations": "Parazynski Port,Tucker Refinery,Dias Survey,Barr Beacon"
+ },
+ {
+ "name": "HIP 14307",
+ "pos_x": 99.5625,
+ "pos_y": -143,
+ "pos_z": -7.8125,
+ "stations": "Pond Orbital,Seyfert Reach,Bulgarin Palace"
+ },
+ {
+ "name": "HIP 14311",
+ "pos_x": 170.8125,
+ "pos_y": -144.84375,
+ "pos_z": 76,
+ "stations": "Rigaux Station,Spielberg Relay,Shajn Station"
+ },
+ {
+ "name": "HIP 14313",
+ "pos_x": 101.0625,
+ "pos_y": -145.1875,
+ "pos_z": -7.96875,
+ "stations": "Riess Hub,Melotte Station,Lindblad Point,Tudela Base"
+ },
+ {
+ "name": "HIP 14361",
+ "pos_x": 125.96875,
+ "pos_y": -226.15625,
+ "pos_z": -51.28125,
+ "stations": "Albumasar Mines,Lee Point,Beriev Mines"
+ },
+ {
+ "name": "HIP 14415",
+ "pos_x": 139.59375,
+ "pos_y": -209.75,
+ "pos_z": -21.1875,
+ "stations": "Vlaicu Base,Dubyago Platform"
+ },
+ {
+ "name": "HIP 14521",
+ "pos_x": 197.0625,
+ "pos_y": -159.78125,
+ "pos_z": 93,
+ "stations": "Stebbins Depot"
+ },
+ {
+ "name": "HIP 14527",
+ "pos_x": 91.875,
+ "pos_y": -142.15625,
+ "pos_z": -19.03125,
+ "stations": "Parkinson Dock,Maunder Port,Thollon's Inheritance"
+ },
+ {
+ "name": "HIP 14532",
+ "pos_x": -61.625,
+ "pos_y": -42.65625,
+ "pos_z": -114.5,
+ "stations": "Vela Installation,Bogdanov Penal colony"
+ },
+ {
+ "name": "HIP 14596",
+ "pos_x": 77.3125,
+ "pos_y": -146.53125,
+ "pos_z": -41.75,
+ "stations": "Check Dock,Otomo Hub,Alexandrov Terminal,Abel Camp"
+ },
+ {
+ "name": "HIP 14611",
+ "pos_x": 124.59375,
+ "pos_y": -166.5625,
+ "pos_z": -2.71875,
+ "stations": "Wolszczan Dock,Ross' Inheritance,Webb Vision,Proctor Hub"
+ },
+ {
+ "name": "HIP 14614",
+ "pos_x": -28.9375,
+ "pos_y": -83.34375,
+ "pos_z": -113.375,
+ "stations": "Rond d'Alembert Vision,Patsayev Depot,Birdseye Holdings"
+ },
+ {
+ "name": "HIP 14617",
+ "pos_x": 130.34375,
+ "pos_y": -191.1875,
+ "pos_z": -18.8125,
+ "stations": "Terry Arsenal"
+ },
+ {
+ "name": "HIP 14619",
+ "pos_x": 151.15625,
+ "pos_y": -164.125,
+ "pos_z": 32,
+ "stations": "Mobius Orbital,Nomen Ring,Janjetov Vision,Brunel Prospect,Metcalf Vision,Bryusov Base,Galois' Claim"
+ },
+ {
+ "name": "HIP 14676",
+ "pos_x": -14,
+ "pos_y": -137.15625,
+ "pos_z": -146.75,
+ "stations": "Morrison Relay,Matheson Lab"
+ },
+ {
+ "name": "HIP 14684",
+ "pos_x": 14.90625,
+ "pos_y": -96.6875,
+ "pos_z": -72.96875,
+ "stations": "Wellman Landing"
+ },
+ {
+ "name": "HIP 14752",
+ "pos_x": 156.09375,
+ "pos_y": -162.46875,
+ "pos_z": 38.46875,
+ "stations": "Brorsen Vision,Turzillo Beacon"
+ },
+ {
+ "name": "HIP 14759",
+ "pos_x": 150.625,
+ "pos_y": -107.09375,
+ "pos_z": 84.46875,
+ "stations": "Brundage Mines,Kirshner Colony,Alten Landing"
+ },
+ {
+ "name": "HIP 14760",
+ "pos_x": 87.0625,
+ "pos_y": -176.375,
+ "pos_z": -60.5,
+ "stations": "Meinel Terminal,Arzachel Dock,Korolyov City,Popper Prospect,Nijland Terminal,Fowler Hub"
+ },
+ {
+ "name": "HIP 14807",
+ "pos_x": -47.4375,
+ "pos_y": -82.9375,
+ "pos_z": -138.78125,
+ "stations": "Hoshi Beacon,Scheerbart Landing,Sheffield Vision"
+ },
+ {
+ "name": "HIP 14836",
+ "pos_x": 155.40625,
+ "pos_y": -209.1875,
+ "pos_z": -8.40625,
+ "stations": "Polikarpov Dock,Malcolm Base"
+ },
+ {
+ "name": "HIP 14857",
+ "pos_x": 67.59375,
+ "pos_y": -121.625,
+ "pos_z": -33.5,
+ "stations": "al-Haytham Point,Bradley Prospect"
+ },
+ {
+ "name": "HIP 1487",
+ "pos_x": 47.5625,
+ "pos_y": -196.40625,
+ "pos_z": 53.625,
+ "stations": "Maskelyne Platform,Delsanti's Claim"
+ },
+ {
+ "name": "HIP 14886",
+ "pos_x": -58.09375,
+ "pos_y": -38.4375,
+ "pos_z": -110.03125,
+ "stations": "Walker Port"
+ },
+ {
+ "name": "HIP 14922",
+ "pos_x": -74.5625,
+ "pos_y": -29.15625,
+ "pos_z": -121.875,
+ "stations": "Liebig Dock,Cayley Gateway,Lavoisier Orbital"
+ },
+ {
+ "name": "HIP 14944",
+ "pos_x": -110.21875,
+ "pos_y": 32.71875,
+ "pos_z": -106.21875,
+ "stations": "Bacon Station,Heinlein Vision,Hilmers Landing,Davidson Installation"
+ },
+ {
+ "name": "HIP 14973",
+ "pos_x": 98.53125,
+ "pos_y": -184.59375,
+ "pos_z": -57.5625,
+ "stations": "Muramatsu City,Thome Terminal,Williams Installation,Heck's Claim,Elder Installation,Sugano City,Walotsky Gateway,Schwarzschild Terminal"
+ },
+ {
+ "name": "HIP 14974",
+ "pos_x": -38.125,
+ "pos_y": -75.75,
+ "pos_z": -122.375,
+ "stations": "Davy Arsenal,Lucid Plant"
+ },
+ {
+ "name": "HIP 14989",
+ "pos_x": 53.75,
+ "pos_y": -148.21875,
+ "pos_z": -78.28125,
+ "stations": "Gehry Terminal,Michell Point"
+ },
+ {
+ "name": "HIP 14997",
+ "pos_x": -26.28125,
+ "pos_y": -114.25,
+ "pos_z": -145.75,
+ "stations": "Charnas Station,Zelazny Hub,Tanaka Horizons"
+ },
+ {
+ "name": "HIP 15046",
+ "pos_x": 13.5,
+ "pos_y": -146.625,
+ "pos_z": -128.53125,
+ "stations": "Stephens Horizons"
+ },
+ {
+ "name": "HIP 15062",
+ "pos_x": -28.53125,
+ "pos_y": -68.34375,
+ "pos_z": -104.0625,
+ "stations": "Deb Vision,Smith Depot"
+ },
+ {
+ "name": "HIP 15205",
+ "pos_x": -71.0625,
+ "pos_y": -54.625,
+ "pos_z": -146.4375,
+ "stations": "Corte-Real Reformatory"
+ },
+ {
+ "name": "HIP 15255",
+ "pos_x": 117.03125,
+ "pos_y": -153.71875,
+ "pos_z": -7.53125,
+ "stations": "De Hub,Zeppelin Dock,Oren Station,Nakano Terminal,Hague Terminal,Rutan Vision,Boscovich Relay,Salpeter Landing,Simak Survey,Merrill Terminal,Hollander Beacon"
+ },
+ {
+ "name": "HIP 15266",
+ "pos_x": -51.4375,
+ "pos_y": -51.875,
+ "pos_z": -119.4375,
+ "stations": "Linnehan Orbital,Rennie Hub"
+ },
+ {
+ "name": "HIP 15278",
+ "pos_x": -51.84375,
+ "pos_y": -83.625,
+ "pos_z": -152.90625,
+ "stations": "Navigator Freeport,Salgari Base"
+ },
+ {
+ "name": "HIP 15279",
+ "pos_x": -14.53125,
+ "pos_y": -137.78125,
+ "pos_z": -160.78125,
+ "stations": "McIntyre Reach"
+ },
+ {
+ "name": "HIP 15286",
+ "pos_x": 35.28125,
+ "pos_y": -103.3125,
+ "pos_z": -61.25,
+ "stations": "Bolton Orbital,Milne Orbital"
+ },
+ {
+ "name": "HIP 15304",
+ "pos_x": -11.84375,
+ "pos_y": -96.40625,
+ "pos_z": -115,
+ "stations": "Westerfeld Orbital,Hennepin Holdings"
+ },
+ {
+ "name": "HIP 15357",
+ "pos_x": 76.90625,
+ "pos_y": -170.90625,
+ "pos_z": -78.625,
+ "stations": "Aaronson Landing,Young Colony,Butcher Arsenal"
+ },
+ {
+ "name": "HIP 1536",
+ "pos_x": 144.875,
+ "pos_y": -214.03125,
+ "pos_z": 110.71875,
+ "stations": "Houten-Groeneveld Survey,Chretien Oudemans Station,Anderson Hub,Meyrink Prospect,Utley Relay"
+ },
+ {
+ "name": "HIP 15362",
+ "pos_x": -12.34375,
+ "pos_y": -130.09375,
+ "pos_z": -151.5625,
+ "stations": "Boulle Holdings"
+ },
+ {
+ "name": "HIP 15374",
+ "pos_x": -16.375,
+ "pos_y": -105.3125,
+ "pos_z": -131.1875,
+ "stations": "Haise Settlement"
+ },
+ {
+ "name": "HIP 15376",
+ "pos_x": 44,
+ "pos_y": -121.5,
+ "pos_z": -69.96875,
+ "stations": "Andoyer Mine"
+ },
+ {
+ "name": "HIP 15381",
+ "pos_x": -34,
+ "pos_y": -85.3125,
+ "pos_z": -133.21875,
+ "stations": "McQuay Landing,Garrett Enterprise,Kelleam Installation"
+ },
+ {
+ "name": "HIP 15415",
+ "pos_x": -93.84375,
+ "pos_y": 36.125,
+ "pos_z": -83.90625,
+ "stations": "Siodmak Camp,Bisson Landing"
+ },
+ {
+ "name": "HIP 15438",
+ "pos_x": 168.34375,
+ "pos_y": -148.34375,
+ "pos_z": 62.6875,
+ "stations": "Adams Dock,Richer Dock,Aikin Landing"
+ },
+ {
+ "name": "HIP 15493",
+ "pos_x": 72.1875,
+ "pos_y": -138.09375,
+ "pos_z": -52.40625,
+ "stations": "van Rhijn Stop,Molchanov Works"
+ },
+ {
+ "name": "HIP 15507",
+ "pos_x": 167,
+ "pos_y": -167,
+ "pos_z": 40.625,
+ "stations": "Nakano Enterprise,Piazzi Station,Penrose Port"
+ },
+ {
+ "name": "HIP 15545",
+ "pos_x": 134.71875,
+ "pos_y": -199,
+ "pos_z": -36.34375,
+ "stations": "Dowty Base,Araki Depot"
+ },
+ {
+ "name": "HIP 15587",
+ "pos_x": 125.6875,
+ "pos_y": -136.875,
+ "pos_z": 17.9375,
+ "stations": "Stebbins Mines,Navigator Settlement,Grushin Orbital,Hiraga's Inheritance"
+ },
+ {
+ "name": "HIP 15604",
+ "pos_x": -107.96875,
+ "pos_y": 23.875,
+ "pos_z": -116.28125,
+ "stations": "Barnaby Enterprise,Asher Terminal,Johnson Orbital"
+ },
+ {
+ "name": "HIP 15609",
+ "pos_x": -47.0625,
+ "pos_y": -41.0625,
+ "pos_z": -106.1875,
+ "stations": "Vasquez de Coronado Colony"
+ },
+ {
+ "name": "HIP 15614",
+ "pos_x": 182.90625,
+ "pos_y": -177.71875,
+ "pos_z": 49,
+ "stations": "Hevelius Hub,Ball Colony,Oikawa Landing,Lloyd Prospect"
+ },
+ {
+ "name": "HIP 15644",
+ "pos_x": 120.21875,
+ "pos_y": -132.46875,
+ "pos_z": 15,
+ "stations": "Brouwer Dock"
+ },
+ {
+ "name": "HIP 15656",
+ "pos_x": 125.09375,
+ "pos_y": -110.75,
+ "pos_z": 44.9375,
+ "stations": "Fuller Arsenal,Furukawa Mine"
+ },
+ {
+ "name": "HIP 15669",
+ "pos_x": -77.0625,
+ "pos_y": -15.59375,
+ "pos_z": -118.9375,
+ "stations": "Oramus Installation,Erdos Vision"
+ },
+ {
+ "name": "HIP 1567",
+ "pos_x": 84.40625,
+ "pos_y": -223.25,
+ "pos_z": 77.375,
+ "stations": "Whittle Station,Trimble Port"
+ },
+ {
+ "name": "HIP 15691",
+ "pos_x": -87.28125,
+ "pos_y": 12.125,
+ "pos_z": -102.375,
+ "stations": "Hawkes' Exile,Blish Stop"
+ },
+ {
+ "name": "HIP 1572",
+ "pos_x": 125.0625,
+ "pos_y": -174.21875,
+ "pos_z": 93.9375,
+ "stations": "Cannon Market,Rayhan al-Biruni Penal colony,Baird Prospect"
+ },
+ {
+ "name": "HIP 1573",
+ "pos_x": -50.625,
+ "pos_y": -132.71875,
+ "pos_z": -11.34375,
+ "stations": "Kuiper Installation,Siddha Vision,Petaja Vision,Saberhagen Arsenal"
+ },
+ {
+ "name": "HIP 15756",
+ "pos_x": 141.15625,
+ "pos_y": -192.15625,
+ "pos_z": -24.21875,
+ "stations": "Wachmann Vision,Stephens Point,Celsius Base"
+ },
+ {
+ "name": "HIP 15815",
+ "pos_x": 97.40625,
+ "pos_y": -203.71875,
+ "pos_z": -96.65625,
+ "stations": "Mobius Works,Marius Survey,Taylor Prospect"
+ },
+ {
+ "name": "HIP 1582",
+ "pos_x": 90.5,
+ "pos_y": -174.40625,
+ "pos_z": 74.21875,
+ "stations": "Trumpler Hub"
+ },
+ {
+ "name": "HIP 15866",
+ "pos_x": 84.03125,
+ "pos_y": -166.0625,
+ "pos_z": -73.53125,
+ "stations": "Messier Prospect,Carpenter Mines"
+ },
+ {
+ "name": "HIP 15868",
+ "pos_x": 38.6875,
+ "pos_y": -127.125,
+ "pos_z": -90.78125,
+ "stations": "Thornycroft Hub,Ponce de Leon Orbital,Brosnan Landing"
+ },
+ {
+ "name": "HIP 15875",
+ "pos_x": -7.75,
+ "pos_y": -98.21875,
+ "pos_z": -120.78125,
+ "stations": "Weber Dock,Babcock Survey"
+ },
+ {
+ "name": "HIP 15886",
+ "pos_x": 190.8125,
+ "pos_y": -157.59375,
+ "pos_z": 79.3125,
+ "stations": "Alcock Prospect,Johnson Colony"
+ },
+ {
+ "name": "HIP 15962",
+ "pos_x": 19.8125,
+ "pos_y": -111.8125,
+ "pos_z": -100.40625,
+ "stations": "Ricardo Gateway,Crouch Depot,Godwin Depot"
+ },
+ {
+ "name": "HIP 16038",
+ "pos_x": 152.46875,
+ "pos_y": -226.59375,
+ "pos_z": -53.46875,
+ "stations": "Hagihara Vision,Sopwith Dock,Artzybasheff Dock,Turzillo Keep,Hedin Terminal"
+ },
+ {
+ "name": "HIP 16064",
+ "pos_x": 61.28125,
+ "pos_y": -144.84375,
+ "pos_z": -83.90625,
+ "stations": "Vess Gateway,Bonestell Vision,Allen St. John City"
+ },
+ {
+ "name": "HIP 16094",
+ "pos_x": 61.3125,
+ "pos_y": -105.25,
+ "pos_z": -38.40625,
+ "stations": "Dilworth Hub,Gentil Station,Khrenov Prospect,Baliunas Survey,Obvious Target"
+ },
+ {
+ "name": "HIP 16114",
+ "pos_x": 134.5625,
+ "pos_y": -162.40625,
+ "pos_z": -5,
+ "stations": "Struve Prospect,Hirasawa Prospect,Longyear Terminal"
+ },
+ {
+ "name": "HIP 16115",
+ "pos_x": 148.96875,
+ "pos_y": -130.125,
+ "pos_z": 52.34375,
+ "stations": "Busemann Orbital,Margulies Penitentiary"
+ },
+ {
+ "name": "HIP 16128",
+ "pos_x": 119.5625,
+ "pos_y": -175.65625,
+ "pos_z": -41.1875,
+ "stations": "Havilland Dock,Oosterhoff City,Ashbrook Dock,Palmer Barracks,Aitken Laboratory"
+ },
+ {
+ "name": "HIP 1613",
+ "pos_x": 77.53125,
+ "pos_y": -185.75,
+ "pos_z": 68.09375,
+ "stations": "Sei Vision"
+ },
+ {
+ "name": "HIP 1625",
+ "pos_x": 4.125,
+ "pos_y": -172.09375,
+ "pos_z": 24.4375,
+ "stations": "Yanai Terminal,Pacheco Dock,Takahashi Installation"
+ },
+ {
+ "name": "HIP 16272",
+ "pos_x": 131.25,
+ "pos_y": -178.59375,
+ "pos_z": -31.375,
+ "stations": "Behnisch Orbital,Stone Port,Amnuel Base,Taine Camp"
+ },
+ {
+ "name": "HIP 16286",
+ "pos_x": 50.9375,
+ "pos_y": -133,
+ "pos_z": -88.59375,
+ "stations": "Bear Keep,Barnard Station,Fadlan Settlement,Gentil Port"
+ },
+ {
+ "name": "HIP 16324",
+ "pos_x": 63.96875,
+ "pos_y": -153.28125,
+ "pos_z": -95.625,
+ "stations": "van den Hove Port,Baade Holdings"
+ },
+ {
+ "name": "HIP 16343",
+ "pos_x": -58.59375,
+ "pos_y": -29.21875,
+ "pos_z": -117.09375,
+ "stations": "Patsayev Port"
+ },
+ {
+ "name": "HIP 16348",
+ "pos_x": 43.96875,
+ "pos_y": -114.5,
+ "pos_z": -77.03125,
+ "stations": "van de Sande Bakhuyzen Survey,Kuchemann City,Dickson Barracks"
+ },
+ {
+ "name": "HIP 16365",
+ "pos_x": 66.03125,
+ "pos_y": -107.90625,
+ "pos_z": -38.4375,
+ "stations": "Maury Vision,Tousey Gateway"
+ },
+ {
+ "name": "HIP 16404",
+ "pos_x": -122.46875,
+ "pos_y": 29,
+ "pos_z": -136.34375,
+ "stations": "Stabenow Beacon"
+ },
+ {
+ "name": "HIP 16405",
+ "pos_x": -34.875,
+ "pos_y": -78.71875,
+ "pos_z": -144.71875,
+ "stations": "Ehrlich Dock,Cabana Enterprise,Titov Dock,Rangarajan Enterprise,Bethke Installation"
+ },
+ {
+ "name": "HIP 16460",
+ "pos_x": 106.40625,
+ "pos_y": -131.0625,
+ "pos_z": -11.125,
+ "stations": "Phillips Platform,Saarinen Survey,Shute's Inheritance"
+ },
+ {
+ "name": "HIP 16477",
+ "pos_x": 25.96875,
+ "pos_y": -112.8125,
+ "pos_z": -102.25,
+ "stations": "Artsutanov Relay,Kibalchich Station"
+ },
+ {
+ "name": "HIP 16523",
+ "pos_x": 151.9375,
+ "pos_y": -163.0625,
+ "pos_z": 12.96875,
+ "stations": "Louis de Lacaille Base,Regiomontanus Settlement"
+ },
+ {
+ "name": "HIP 16529",
+ "pos_x": -34.21875,
+ "pos_y": -61.375,
+ "pos_z": -124.5625,
+ "stations": "Yourkevitch Port"
+ },
+ {
+ "name": "HIP 16538",
+ "pos_x": -24.625,
+ "pos_y": -84.0625,
+ "pos_z": -139.34375,
+ "stations": "Gaffney Dock,Ampere Enterprise,Bennett City"
+ },
+ {
+ "name": "HIP 16540",
+ "pos_x": 117.46875,
+ "pos_y": -112.4375,
+ "pos_z": 26.78125,
+ "stations": "DiFate Installation,Stebbins Depot"
+ },
+ {
+ "name": "HIP 16591",
+ "pos_x": -77.84375,
+ "pos_y": -39.8125,
+ "pos_z": -160.59375,
+ "stations": "Dukaj Mines,Verrazzano Point"
+ },
+ {
+ "name": "HIP 16595",
+ "pos_x": 127.40625,
+ "pos_y": -170.96875,
+ "pos_z": -32.75,
+ "stations": "Schroeder Town,Parker Dock,Francis Survey,Ptack Vision,Quinn Plant"
+ },
+ {
+ "name": "HIP 16607",
+ "pos_x": 42.59375,
+ "pos_y": -125.28125,
+ "pos_z": -96.46875,
+ "stations": "Thome Gateway,Haro Station,Bell Port,Dahm Observatory,Couper Survey"
+ },
+ {
+ "name": "HIP 16646",
+ "pos_x": -108.8125,
+ "pos_y": 39.59375,
+ "pos_z": -105.53125,
+ "stations": "Henderson's Claim,Bradley Penitentiary"
+ },
+ {
+ "name": "HIP 16712",
+ "pos_x": -106.03125,
+ "pos_y": 11.59375,
+ "pos_z": -137.5625,
+ "stations": "Coats Beacon,Borman Installation,Vesalius Beacon"
+ },
+ {
+ "name": "HIP 1672",
+ "pos_x": 142.34375,
+ "pos_y": -230.8125,
+ "pos_z": 110.375,
+ "stations": "Wallis Stop"
+ },
+ {
+ "name": "HIP 16723",
+ "pos_x": -0.4375,
+ "pos_y": -120,
+ "pos_z": -153.6875,
+ "stations": "Soper Plant"
+ },
+ {
+ "name": "HIP 16727",
+ "pos_x": 13.375,
+ "pos_y": -109.3125,
+ "pos_z": -120.3125,
+ "stations": "Whitney Ring,Back Relay,Forward Enterprise"
+ },
+ {
+ "name": "HIP 16747",
+ "pos_x": -42.21875,
+ "pos_y": -30.96875,
+ "pos_z": -100.4375,
+ "stations": "Hill Penitentiary,Mitchell Legacy,Webb Station"
+ },
+ {
+ "name": "HIP 16753",
+ "pos_x": -88.3125,
+ "pos_y": -172.9375,
+ "pos_z": -348.84375,
+ "stations": "Goya Landing,Liman Legacy"
+ },
+ {
+ "name": "HIP 16783",
+ "pos_x": 54.21875,
+ "pos_y": -117.34375,
+ "pos_z": -72.78125,
+ "stations": "Finlay Orbital"
+ },
+ {
+ "name": "HIP 16813",
+ "pos_x": -57.03125,
+ "pos_y": -143.375,
+ "pos_z": -268.28125,
+ "stations": "Sisters' Refuge"
+ },
+ {
+ "name": "HIP 16858",
+ "pos_x": 60.34375,
+ "pos_y": -115.03125,
+ "pos_z": -62.0625,
+ "stations": "Hansen Port,Shirazi Enterprise,Thierree Station,Kozin Barracks"
+ },
+ {
+ "name": "HIP 1687",
+ "pos_x": -122.5,
+ "pos_y": -119.0625,
+ "pos_z": -55.25,
+ "stations": "Green Horizons"
+ },
+ {
+ "name": "HIP 16914",
+ "pos_x": 145.1875,
+ "pos_y": -121.0625,
+ "pos_z": 53.125,
+ "stations": "Wilhelm von Struve City,Schiaparelli Base,Bradfield Gateway,Amedeo Plana Lab,Russell Terminal"
+ },
+ {
+ "name": "HIP 16933",
+ "pos_x": 15.875,
+ "pos_y": -95.3125,
+ "pos_z": -102.40625,
+ "stations": "Wiberg Settlement,Cockrell Colony,Penn Prospect"
+ },
+ {
+ "name": "HIP 16954",
+ "pos_x": 114.1875,
+ "pos_y": -177.84375,
+ "pos_z": -67.5625,
+ "stations": "Plait Hub,Kotelnikov Station,Zarnecki Hub,Archer Depot"
+ },
+ {
+ "name": "HIP 16959",
+ "pos_x": 165.40625,
+ "pos_y": -176.09375,
+ "pos_z": 9.75,
+ "stations": "Young Enterprise,Espenak Dock,Gurshtein Prospect"
+ },
+ {
+ "name": "HIP 16984",
+ "pos_x": 155.0625,
+ "pos_y": -205.125,
+ "pos_z": -44.28125,
+ "stations": "Vyssotsky Vision"
+ },
+ {
+ "name": "HIP 17022",
+ "pos_x": -58.1875,
+ "pos_y": -22.5625,
+ "pos_z": -115.90625,
+ "stations": "Dutton Terminal,Bluford Gateway,Buffett Ring,Steele Depot,Altman Dock,Good Ring,Bova Holdings"
+ },
+ {
+ "name": "HIP 17044",
+ "pos_x": -74.25,
+ "pos_y": -129.53125,
+ "pos_z": -283.15625,
+ "stations": "Dionysus"
+ },
+ {
+ "name": "HIP 17047",
+ "pos_x": 110.59375,
+ "pos_y": -154.53125,
+ "pos_z": -43.625,
+ "stations": "Nomen Survey,Weaver Colony"
+ },
+ {
+ "name": "HIP 17108",
+ "pos_x": 84.71875,
+ "pos_y": -155.875,
+ "pos_z": -84.75,
+ "stations": "Paola Station,Garrett Works"
+ },
+ {
+ "name": "HIP 17118",
+ "pos_x": -87.4375,
+ "pos_y": 17.46875,
+ "pos_z": -106.21875,
+ "stations": "King Relay,Scott Freeport,Vries Landing,Wilhelm Installation"
+ },
+ {
+ "name": "HIP 17126",
+ "pos_x": -83.625,
+ "pos_y": 16.71875,
+ "pos_z": -101.625,
+ "stations": "Plante Terminal,Bertin Enterprise"
+ },
+ {
+ "name": "HIP 17171",
+ "pos_x": 163.09375,
+ "pos_y": -188.875,
+ "pos_z": -14.125,
+ "stations": "Arnold Oasis,Youll Colony,Haldeman II Bastion"
+ },
+ {
+ "name": "HIP 17175",
+ "pos_x": 111.6875,
+ "pos_y": -192.25,
+ "pos_z": -95.46875,
+ "stations": "Nakamura Relay"
+ },
+ {
+ "name": "HIP 17225",
+ "pos_x": -86.15625,
+ "pos_y": -165.59375,
+ "pos_z": -356.8125,
+ "stations": "PRE Research Base"
+ },
+ {
+ "name": "HIP 17269",
+ "pos_x": 171.8125,
+ "pos_y": -185.21875,
+ "pos_z": 2.21875,
+ "stations": "Morrow Holdings,Pittendreigh Landing,Coelho Installation"
+ },
+ {
+ "name": "HIP 17272",
+ "pos_x": 14.28125,
+ "pos_y": -108.46875,
+ "pos_z": -128.59375,
+ "stations": "Zebrowski Orbital,Zahn Penal colony,Herndon Hub"
+ },
+ {
+ "name": "HIP 17276",
+ "pos_x": 50.375,
+ "pos_y": -99.65625,
+ "pos_z": -62.1875,
+ "stations": "Bloomfield Port,Aitken Point,Yolen Installation"
+ },
+ {
+ "name": "HIP 17298",
+ "pos_x": 78.84375,
+ "pos_y": -102.59375,
+ "pos_z": -23.5625,
+ "stations": "Crown Base,Ayton Innes Vision,Louis de Lacaille Terminal,Wheeler Landing,Froud Hub,Ptack Dock,Chiang Enterprise"
+ },
+ {
+ "name": "HIP 17305",
+ "pos_x": -35.25,
+ "pos_y": -72.53125,
+ "pos_z": -153.9375,
+ "stations": "Blackwell Landing,Schmitt Escape"
+ },
+ {
+ "name": "HIP 1732",
+ "pos_x": -23.46875,
+ "pos_y": -192.375,
+ "pos_z": 9.90625,
+ "stations": "Esposito Dock,Gould Hub,Fozard Vision,Narbeth Plant"
+ },
+ {
+ "name": "HIP 1736",
+ "pos_x": -126.75,
+ "pos_y": -139.6875,
+ "pos_z": -55.90625,
+ "stations": "Belyanin Vision,Timofeyevich Station,Bendell Dock,Siegel Forum"
+ },
+ {
+ "name": "HIP 17412",
+ "pos_x": -71.25,
+ "pos_y": -41.59375,
+ "pos_z": -167.625,
+ "stations": "Tepper Prospect,Corte-Real Installation"
+ },
+ {
+ "name": "HIP 1742",
+ "pos_x": 29.1875,
+ "pos_y": -244.46875,
+ "pos_z": 46.28125,
+ "stations": "Quick Enterprise,Davies Vision,Gurney Port,Brahmagupta Dock,King Enterprise"
+ },
+ {
+ "name": "HIP 1745",
+ "pos_x": -16.09375,
+ "pos_y": -174.90625,
+ "pos_z": 11.84375,
+ "stations": "Ilyushin Vision,Anthony Terminal"
+ },
+ {
+ "name": "HIP 17478",
+ "pos_x": 39.90625,
+ "pos_y": -120.15625,
+ "pos_z": -110.78125,
+ "stations": "Qureshi Camp,Giancola Mine"
+ },
+ {
+ "name": "HIP 17483",
+ "pos_x": 78.90625,
+ "pos_y": -114,
+ "pos_z": -42.15625,
+ "stations": "Isaev Vision,Giacobini Vision,Artsutanov Station,Goryu Base"
+ },
+ {
+ "name": "HIP 17486",
+ "pos_x": 130.59375,
+ "pos_y": -115.84375,
+ "pos_z": 34.5,
+ "stations": "de Kamp Hub,Faye Dock,Cole Prospect,Kulin Vision,Lassell Vision,Joseph Delambre Terminal,Eddington Hub,Bachman Beacon,Eggleton Hub,Schulhof Dock,Vuia Terminal,Alexander Arena,Wegener Point"
+ },
+ {
+ "name": "HIP 17497",
+ "pos_x": -79.125,
+ "pos_y": -158.6875,
+ "pos_z": -349.125,
+ "stations": "Bao Landing"
+ },
+ {
+ "name": "HIP 17515",
+ "pos_x": 125.3125,
+ "pos_y": -111.0625,
+ "pos_z": 33.0625,
+ "stations": "Fabricius Terminal,Osterbrock City,Gurshtein Hub"
+ },
+ {
+ "name": "HIP 17519",
+ "pos_x": 17.375,
+ "pos_y": -160.84375,
+ "pos_z": -204.5,
+ "stations": "Pirate's Lament"
+ },
+ {
+ "name": "HIP 17568",
+ "pos_x": -104.34375,
+ "pos_y": 11.53125,
+ "pos_z": -144.53125,
+ "stations": "Coelho Terminal"
+ },
+ {
+ "name": "HIP 17621",
+ "pos_x": 55,
+ "pos_y": -110.40625,
+ "pos_z": -76.0625,
+ "stations": "Bus Station,Fleming Enterprise"
+ },
+ {
+ "name": "HIP 17655",
+ "pos_x": -83.40625,
+ "pos_y": -32.90625,
+ "pos_z": -178.375,
+ "stations": "Nehsi Landing"
+ },
+ {
+ "name": "HIP 17675",
+ "pos_x": -96.84375,
+ "pos_y": -5.78125,
+ "pos_z": -159.78125,
+ "stations": "Tem Oasis"
+ },
+ {
+ "name": "HIP 17692",
+ "pos_x": -74.125,
+ "pos_y": -143.96875,
+ "pos_z": -328.78125,
+ "stations": "Blackmount Habitation,Blackmount Orbital"
+ },
+ {
+ "name": "HIP 17694",
+ "pos_x": -72.25,
+ "pos_y": -152.53125,
+ "pos_z": -338.6875,
+ "stations": "Hudson Observatory"
+ },
+ {
+ "name": "HIP 17706",
+ "pos_x": -93.375,
+ "pos_y": 0.03125,
+ "pos_z": -146.125,
+ "stations": "Oltion Station,Smith Silo,Swanwick Platform"
+ },
+ {
+ "name": "HIP 1771",
+ "pos_x": -79.40625,
+ "pos_y": -123.25,
+ "pos_z": -31.03125,
+ "stations": "Scott Horizons"
+ },
+ {
+ "name": "HIP 17719",
+ "pos_x": -46.46875,
+ "pos_y": -23.9375,
+ "pos_z": -108.34375,
+ "stations": "Fawcett's Pride"
+ },
+ {
+ "name": "HIP 17736",
+ "pos_x": -83.5,
+ "pos_y": -3.09375,
+ "pos_z": -135.6875,
+ "stations": "Malaspina Keep"
+ },
+ {
+ "name": "HIP 17742",
+ "pos_x": 102.78125,
+ "pos_y": -159.40625,
+ "pos_z": -76.125,
+ "stations": "Iben Dock,Anderson Horizons,Heyerdahl Relay,Gann Point"
+ },
+ {
+ "name": "HIP 17751",
+ "pos_x": 136.375,
+ "pos_y": -176.40625,
+ "pos_z": -48.8125,
+ "stations": "Ruppelt Vision"
+ },
+ {
+ "name": "HIP 17766",
+ "pos_x": 1.96875,
+ "pos_y": -69.125,
+ "pos_z": -100.1875,
+ "stations": "Ray Installation,Knight Settlement"
+ },
+ {
+ "name": "HIP 17782",
+ "pos_x": -87.53125,
+ "pos_y": -4.125,
+ "pos_z": -144.03125,
+ "stations": "Griffith's Inheritance,Godel Settlement,Pryor Holdings"
+ },
+ {
+ "name": "HIP 17790",
+ "pos_x": -89.5625,
+ "pos_y": 8.40625,
+ "pos_z": -128.5625,
+ "stations": "McCarthy Settlement,Navigator Works"
+ },
+ {
+ "name": "HIP 1781",
+ "pos_x": 91.625,
+ "pos_y": -263.65625,
+ "pos_z": 83.96875,
+ "stations": "Chretien Oudemans Port,Riemann's Folly,Jansky Survey,van de Sande Bakhuyzen Ring"
+ },
+ {
+ "name": "HIP 17819",
+ "pos_x": 100.375,
+ "pos_y": -137.40625,
+ "pos_z": -48.28125,
+ "stations": "Pangborn Vision,Gaensler Survey,Jackson Terminal"
+ },
+ {
+ "name": "HIP 17838",
+ "pos_x": 68.96875,
+ "pos_y": -123.59375,
+ "pos_z": -77.4375,
+ "stations": "Arend Dock,Francis Port,Dittmar Terminal"
+ },
+ {
+ "name": "HIP 17892",
+ "pos_x": -70.75,
+ "pos_y": -157.9375,
+ "pos_z": -352.84375,
+ "stations": "Kamov Survey,Rescue Ship - Kamov Survey"
+ },
+ {
+ "name": "HIP 17903",
+ "pos_x": 45.28125,
+ "pos_y": -112.28125,
+ "pos_z": -99,
+ "stations": "Lalande Enterprise,Tomita Vision,Whelan Prospect"
+ },
+ {
+ "name": "HIP 1795",
+ "pos_x": 32.125,
+ "pos_y": -248.0625,
+ "pos_z": 47.6875,
+ "stations": "Serrao Beacon,Schiaparelli Oasis,Zarnecki Terminal"
+ },
+ {
+ "name": "HIP 17962",
+ "pos_x": -15.78125,
+ "pos_y": -66.0625,
+ "pos_z": -126.71875,
+ "stations": "MacLean Keep"
+ },
+ {
+ "name": "HIP 17970",
+ "pos_x": 133.53125,
+ "pos_y": -127.25,
+ "pos_z": 17.9375,
+ "stations": "Marcy Dock"
+ },
+ {
+ "name": "HIP 17984",
+ "pos_x": 160.03125,
+ "pos_y": -208.0625,
+ "pos_z": -64.25,
+ "stations": "Messerschmitt Survey,Bohrmann Horizons,Serling Prospect,Gloss Relay"
+ },
+ {
+ "name": "HIP 18011",
+ "pos_x": 144.9375,
+ "pos_y": -172.53125,
+ "pos_z": -34.25,
+ "stations": "Fox Station,Herschel Colony,Flaugergues Horizons"
+ },
+ {
+ "name": "HIP 18075",
+ "pos_x": 104.46875,
+ "pos_y": -172.09375,
+ "pos_z": -100.34375,
+ "stations": "Condit Depot,Svavarsson Relay"
+ },
+ {
+ "name": "HIP 18077",
+ "pos_x": -303.84375,
+ "pos_y": -236.125,
+ "pos_z": -860.59375,
+ "stations": "Mahon Research Base"
+ },
+ {
+ "name": "HIP 18150",
+ "pos_x": 112.46875,
+ "pos_y": -157.4375,
+ "pos_z": -66.1875,
+ "stations": "Wachmann Port,Malvern Landing,Baffin Silo"
+ },
+ {
+ "name": "HIP 18187",
+ "pos_x": 78.21875,
+ "pos_y": -102.53125,
+ "pos_z": -35.6875,
+ "stations": "Tuttle Gateway,Tennyson d'Eyncourt Depot"
+ },
+ {
+ "name": "HIP 18218",
+ "pos_x": -88.71875,
+ "pos_y": 0.1875,
+ "pos_z": -145.09375,
+ "stations": "Alvares Dock,Rayhan al-Biruni Dock,Harper Survey"
+ },
+ {
+ "name": "HIP 18305",
+ "pos_x": 102.0625,
+ "pos_y": -144.875,
+ "pos_z": -66.59375,
+ "stations": "Evans Platform,Curtiss Port,Sinisalo Holdings,Connes Holdings"
+ },
+ {
+ "name": "HIP 18322",
+ "pos_x": -4.40625,
+ "pos_y": -63.3125,
+ "pos_z": -110.375,
+ "stations": "Fisher Plant,Hudson Vision,Kolmogorov Arsenal,Kelly Platform"
+ },
+ {
+ "name": "HIP 18327",
+ "pos_x": -12.8125,
+ "pos_y": -61.46875,
+ "pos_z": -121.4375,
+ "stations": "Stevens Orbital,Caillie Hub,Narita Ring,Erikson Base,Wagner Barracks"
+ },
+ {
+ "name": "HIP 18339",
+ "pos_x": 45.46875,
+ "pos_y": -111.28125,
+ "pos_z": -106.375,
+ "stations": "Broglie Gateway,Manarov Ring,Griffith Hub,Oramus Escape"
+ },
+ {
+ "name": "HIP 18387",
+ "pos_x": 131.09375,
+ "pos_y": -120.71875,
+ "pos_z": 19.8125,
+ "stations": "Gunter Installation"
+ },
+ {
+ "name": "HIP 18388",
+ "pos_x": 30.6875,
+ "pos_y": -103.875,
+ "pos_z": -119.78125,
+ "stations": "Karl Diesel Depot"
+ },
+ {
+ "name": "HIP 18402",
+ "pos_x": 119.34375,
+ "pos_y": -139.6875,
+ "pos_z": -31.34375,
+ "stations": "Ortiz Moreno Landing"
+ },
+ {
+ "name": "HIP 18420",
+ "pos_x": 169.59375,
+ "pos_y": -210.5625,
+ "pos_z": -64.90625,
+ "stations": "Vess Depot"
+ },
+ {
+ "name": "HIP 18427",
+ "pos_x": 125.21875,
+ "pos_y": -136.125,
+ "pos_z": -16.03125,
+ "stations": "Amedeo Plana Vision,Nadiradze Gateway,Roth Dock,Gent Legacy,Alcock Hub"
+ },
+ {
+ "name": "HIP 18466",
+ "pos_x": 113.1875,
+ "pos_y": -136.3125,
+ "pos_z": -37.03125,
+ "stations": "d'Arrest Dock,Wood Depot,Harrison Vision"
+ },
+ {
+ "name": "HIP 18496",
+ "pos_x": 161.375,
+ "pos_y": -197.5,
+ "pos_z": -58.9375,
+ "stations": "Plaskett Vision"
+ },
+ {
+ "name": "HIP 18497",
+ "pos_x": 110.40625,
+ "pos_y": -135.15625,
+ "pos_z": -40.34375,
+ "stations": "Tevis Camp,Fedden Escape"
+ },
+ {
+ "name": "HIP 18518",
+ "pos_x": -61.5625,
+ "pos_y": -39.15625,
+ "pos_z": -169.90625,
+ "stations": "Tepper Bastion"
+ },
+ {
+ "name": "HIP 18527",
+ "pos_x": 46.71875,
+ "pos_y": -87.6875,
+ "pos_z": -68.8125,
+ "stations": "Zacuto Ring,Gurney Platform,Killough Relay,Eddington Orbital"
+ },
+ {
+ "name": "HIP 18534",
+ "pos_x": 135.65625,
+ "pos_y": -160.34375,
+ "pos_z": -41.09375,
+ "stations": "Condit Colony,Berkey Colony,Key Plant,Rice Holdings,Brooks Vision"
+ },
+ {
+ "name": "HIP 18563",
+ "pos_x": 156,
+ "pos_y": -196.25,
+ "pos_z": -68.5,
+ "stations": "Pilcher Survey"
+ },
+ {
+ "name": "HIP 18609",
+ "pos_x": -15.96875,
+ "pos_y": -85.65625,
+ "pos_z": -173.90625,
+ "stations": "Kessel Legacy,Willis Relay,Wisniewski-Snerg Plant"
+ },
+ {
+ "name": "HIP 1866",
+ "pos_x": -60.46875,
+ "pos_y": -139.78125,
+ "pos_z": -19.09375,
+ "stations": "Gulyaev City,Lem Colony,Lynn Colony,Baillaud Relay,Rond d'Alembert's Claim"
+ },
+ {
+ "name": "HIP 18702",
+ "pos_x": 146.6875,
+ "pos_y": -190.15625,
+ "pos_z": -78.46875,
+ "stations": "Meech Base"
+ },
+ {
+ "name": "HIP 18707",
+ "pos_x": 76.25,
+ "pos_y": -105.34375,
+ "pos_z": -52.15625,
+ "stations": "Mueller Port,Polya Installation,Slade Point"
+ },
+ {
+ "name": "HIP 1871",
+ "pos_x": -121.65625,
+ "pos_y": -46.75,
+ "pos_z": -65.125,
+ "stations": "Bova Enterprise,Gresh Hub,Ostrander Mines"
+ },
+ {
+ "name": "HIP 18713",
+ "pos_x": 144.09375,
+ "pos_y": -156.84375,
+ "pos_z": -24.9375,
+ "stations": "Dubyago Settlement,Prandtl Horizons"
+ },
+ {
+ "name": "HIP 18714",
+ "pos_x": 95.53125,
+ "pos_y": -118.75,
+ "pos_z": -42.34375,
+ "stations": "Jackson Port,Lange Silo"
+ },
+ {
+ "name": "HIP 18725",
+ "pos_x": -76.3125,
+ "pos_y": 4.78125,
+ "pos_z": -123.78125,
+ "stations": "Lessing Works,Potagos Terminal,Merchiston Station,Stjepan Seljan Enterprise,Stross Settlement,Wallis Enterprise,Gessi City"
+ },
+ {
+ "name": "HIP 18828",
+ "pos_x": 77.03125,
+ "pos_y": -102.28125,
+ "pos_z": -47.53125,
+ "stations": "Gorgani Ring,Ruppelt City,Chadbourne Hub"
+ },
+ {
+ "name": "HIP 18839",
+ "pos_x": 97.65625,
+ "pos_y": -147.84375,
+ "pos_z": -93.3125,
+ "stations": "Luu Terminal,van de Sande Bakhuyzen Vision,Hanke-Woods' Inheritance"
+ },
+ {
+ "name": "HIP 18843",
+ "pos_x": -9.5625,
+ "pos_y": -87.40625,
+ "pos_z": -173.5,
+ "stations": "Alexander Freeport,Bentham Platform,Windt Town,Cummings Survey,Dampier Settlement"
+ },
+ {
+ "name": "HIP 1885",
+ "pos_x": 12.03125,
+ "pos_y": -128.71875,
+ "pos_z": 21.53125,
+ "stations": "Nijland Station,Bartolomeu de Gusmao Lab,Wasden Orbital,Wegner Station,Bode Arsenal"
+ },
+ {
+ "name": "HIP 18857",
+ "pos_x": 135.0625,
+ "pos_y": -174,
+ "pos_z": -74.84375,
+ "stations": "Morrill Station,Kuchner Platform,Younghusband Horizons,Leberecht Tempel Port"
+ },
+ {
+ "name": "HIP 18870",
+ "pos_x": 91.46875,
+ "pos_y": -140.71875,
+ "pos_z": -92.09375,
+ "stations": "Carrington Vision,Humphreys Landing,Jordan Laboratory"
+ },
+ {
+ "name": "HIP 18888",
+ "pos_x": 129.21875,
+ "pos_y": -152.40625,
+ "pos_z": -47.0625,
+ "stations": "Kosai Prospect,Kurtz Holdings,Sorayama Prospect"
+ },
+ {
+ "name": "HIP 18918",
+ "pos_x": 58.84375,
+ "pos_y": -98.59375,
+ "pos_z": -74.65625,
+ "stations": "Johnson Ring,Sikorsky Dock"
+ },
+ {
+ "name": "HIP 18946",
+ "pos_x": -14.4375,
+ "pos_y": -56.03125,
+ "pos_z": -127.6875,
+ "stations": "Krupkat Vision,Ahmed Horizons,Frobisher Station,Dall's Pride"
+ },
+ {
+ "name": "HIP 18958",
+ "pos_x": 142.1875,
+ "pos_y": -166.71875,
+ "pos_z": -51.5,
+ "stations": "Muller Terminal,Morrison Hub"
+ },
+ {
+ "name": "HIP 18964",
+ "pos_x": 173.03125,
+ "pos_y": -163.53125,
+ "pos_z": 9.0625,
+ "stations": "Schomburg's Claim"
+ },
+ {
+ "name": "HIP 19007",
+ "pos_x": 33.375,
+ "pos_y": -95.875,
+ "pos_z": -116.6875,
+ "stations": "Ellern Settlement"
+ },
+ {
+ "name": "HIP 19052",
+ "pos_x": 115.1875,
+ "pos_y": -152.53125,
+ "pos_z": -75.90625,
+ "stations": "August von Steinheil Enterprise,Ptack Port"
+ },
+ {
+ "name": "HIP 19072",
+ "pos_x": -54.28125,
+ "pos_y": -121.03125,
+ "pos_z": -323.0625,
+ "stations": "Cooper Research Centre"
+ },
+ {
+ "name": "HIP 19091",
+ "pos_x": 116.875,
+ "pos_y": -164.96875,
+ "pos_z": -97.5625,
+ "stations": "Canty Orbital,Selberg Depot,McCrea City,Boyer Terminal"
+ },
+ {
+ "name": "HIP 1914",
+ "pos_x": 142.78125,
+ "pos_y": -154.96875,
+ "pos_z": 100.125,
+ "stations": "Kemurdzhian Terminal,Tilley Installation,Thorne Orbital,Narbeth Arsenal,Kempf Vision"
+ },
+ {
+ "name": "HIP 19147",
+ "pos_x": 10.34375,
+ "pos_y": -105.84375,
+ "pos_z": -180.8125,
+ "stations": "Bartlett Landing,Priest Survey,Mitchell Base"
+ },
+ {
+ "name": "HIP 19148",
+ "pos_x": -6.90625,
+ "pos_y": -64.84375,
+ "pos_z": -134.90625,
+ "stations": "Davis Prospect,Boswell Platform,Lindsey Hangar"
+ },
+ {
+ "name": "HIP 19157",
+ "pos_x": -5.40625,
+ "pos_y": -84.25,
+ "pos_z": -169.09375,
+ "stations": "MacLeod Horizons,Farmer Orbital,Cook Dock,Robinson Holdings"
+ },
+ {
+ "name": "HIP 19188",
+ "pos_x": 172.71875,
+ "pos_y": -124.21875,
+ "pos_z": 79.0625,
+ "stations": "Danforth Platform,Lindemann Vision,Hirayama Works"
+ },
+ {
+ "name": "HIP 19198",
+ "pos_x": 117.75,
+ "pos_y": -158.5625,
+ "pos_z": -86.78125,
+ "stations": "Grego Survey,Comper Dock"
+ },
+ {
+ "name": "HIP 19212",
+ "pos_x": 126.34375,
+ "pos_y": -144.28125,
+ "pos_z": -44.3125,
+ "stations": "Knorre Vision,Regiomontanus Orbital,Armero Oasis,Rice Arsenal"
+ },
+ {
+ "name": "HIP 19213",
+ "pos_x": 172.1875,
+ "pos_y": -193.1875,
+ "pos_z": -53.84375,
+ "stations": "Corbusier Survey,Kirk Depot,Macedo Prospect,Cassini Depot"
+ },
+ {
+ "name": "HIP 19232",
+ "pos_x": 71.15625,
+ "pos_y": -105.21875,
+ "pos_z": -71.09375,
+ "stations": "Koolhaas Settlement"
+ },
+ {
+ "name": "HIP 19257",
+ "pos_x": 5.53125,
+ "pos_y": -67.6875,
+ "pos_z": -120,
+ "stations": "Ivanov Hub,Drzewiecki Hub"
+ },
+ {
+ "name": "HIP 19316",
+ "pos_x": 0.8125,
+ "pos_y": -58.15625,
+ "pos_z": -111.5625,
+ "stations": "Lorenz Dock"
+ },
+ {
+ "name": "HIP 19327",
+ "pos_x": 115.90625,
+ "pos_y": -112.46875,
+ "pos_z": -4.1875,
+ "stations": "Xuesen Station,Alfven Gateway,Tokubei Vision"
+ },
+ {
+ "name": "HIP 1933",
+ "pos_x": -13.625,
+ "pos_y": -153.0625,
+ "pos_z": 9.03125,
+ "stations": "Shumil Base,Kirshner Vision"
+ },
+ {
+ "name": "HIP 19331",
+ "pos_x": 112.875,
+ "pos_y": -126.28125,
+ "pos_z": -36.78125,
+ "stations": "Ahnert Market,Karman Dock,Penrose Hub,Longomontanus Port,Fallows Station,Plancius Vision,Emshwiller Barracks,Kuiper Landing,Hamilton Vision"
+ },
+ {
+ "name": "HIP 19334",
+ "pos_x": 145.25,
+ "pos_y": -151.25,
+ "pos_z": -25.40625,
+ "stations": "Leonard Survey,Osterbrock Oasis,Dowie Oasis,Toll Holdings"
+ },
+ {
+ "name": "HIP 1937",
+ "pos_x": 137.5625,
+ "pos_y": -135.71875,
+ "pos_z": 94.90625,
+ "stations": "Pelliot Gateway,Gunter Port,Le Guin Vision,Whelan Station,Johnson Prospect"
+ },
+ {
+ "name": "HIP 19408",
+ "pos_x": -113.75,
+ "pos_y": 19.875,
+ "pos_z": -173.1875,
+ "stations": "Brackett Point,Klein Retreat,Gunn Base,Witt Observatory"
+ },
+ {
+ "name": "HIP 19410",
+ "pos_x": 51.25,
+ "pos_y": -96.75,
+ "pos_z": -94.96875,
+ "stations": "Malzberg Asylum"
+ },
+ {
+ "name": "HIP 19428",
+ "pos_x": -32.53125,
+ "pos_y": -32.1875,
+ "pos_z": -124.46875,
+ "stations": "Burbank Ring,Fontana Gateway,Adams Base,Nielsen Horizons"
+ },
+ {
+ "name": "HIP 19442",
+ "pos_x": 132.59375,
+ "pos_y": -139.875,
+ "pos_z": -28.625,
+ "stations": "Jones Terminal,Abbot Station,Chernykh Terminal,Bouwens Terminal,Goldstein Town,Parker Station,Metcalf Terminal,Narlikar Port,Barbosa's Inheritance"
+ },
+ {
+ "name": "HIP 19510",
+ "pos_x": 60.65625,
+ "pos_y": -101.59375,
+ "pos_z": -89.34375,
+ "stations": "Schlegel Platform,Maudslay Port,Butz Base"
+ },
+ {
+ "name": "HIP 19582",
+ "pos_x": 109.5625,
+ "pos_y": -132.6875,
+ "pos_z": -61.21875,
+ "stations": "Otus Stop,Suntzeff Asylum"
+ },
+ {
+ "name": "HIP 19591",
+ "pos_x": -16.9375,
+ "pos_y": -41.09375,
+ "pos_z": -116.21875,
+ "stations": "Carey Terminal,Onufrienko Point"
+ },
+ {
+ "name": "HIP 19684",
+ "pos_x": 99.625,
+ "pos_y": -137.75,
+ "pos_z": -93.0625,
+ "stations": "Ban Port,Jackson Observatory,Geller Platform"
+ },
+ {
+ "name": "HIP 19757",
+ "pos_x": 10.3125,
+ "pos_y": -82.3125,
+ "pos_z": -153.125,
+ "stations": "Brunel Landing"
+ },
+ {
+ "name": "HIP 19758",
+ "pos_x": 97.71875,
+ "pos_y": -105.46875,
+ "pos_z": -31.4375,
+ "stations": "Melotte Terminal,Kranz Orbital,Finch Installation"
+ },
+ {
+ "name": "HIP 1976",
+ "pos_x": 0.15625,
+ "pos_y": -152.46875,
+ "pos_z": 16.5,
+ "stations": "Theodorsen's Progress,Kanai Settlement,Schoenherr Horizons,Boss Terminal,Wheeler Mines"
+ },
+ {
+ "name": "HIP 19767",
+ "pos_x": 2.5,
+ "pos_y": -54.09375,
+ "pos_z": -109.28125,
+ "stations": "Panshin Orbital,Isherwood Prospect,Benyovszky Ring,Tarski Relay,Kozin Port,Lundwall Orbital,Greenland Laboratory"
+ },
+ {
+ "name": "HIP 1977",
+ "pos_x": 83.375,
+ "pos_y": -246.3125,
+ "pos_z": 75.09375,
+ "stations": "Fox Terminal,Adams Mines,Dowie Depot"
+ },
+ {
+ "name": "HIP 19773",
+ "pos_x": 185.25,
+ "pos_y": -160.4375,
+ "pos_z": 23.3125,
+ "stations": "Furukawa Colony"
+ },
+ {
+ "name": "HIP 19776",
+ "pos_x": 187.28125,
+ "pos_y": -153.625,
+ "pos_z": 41.625,
+ "stations": "Oort Depot,Hencke Camp"
+ },
+ {
+ "name": "HIP 1978",
+ "pos_x": -114.9375,
+ "pos_y": -39.3125,
+ "pos_z": -62.78125,
+ "stations": "al-Din Plant,Ramaswamy Arsenal"
+ },
+ {
+ "name": "HIP 19781",
+ "pos_x": -1.46875,
+ "pos_y": -63.40625,
+ "pos_z": -136.90625,
+ "stations": "Broglie Settlement,Merbold Outpost"
+ },
+ {
+ "name": "HIP 19786",
+ "pos_x": 2.75,
+ "pos_y": -61.9375,
+ "pos_z": -125.59375,
+ "stations": "Wingrove Terminal,Bryusov Terminal,Brendan Dock,Tennyson d'Eyncourt Station,Okorafor Dock,Russo Terminal,Hume Orbital"
+ },
+ {
+ "name": "HIP 19796",
+ "pos_x": 6.34375,
+ "pos_y": -67.4375,
+ "pos_z": -130.40625,
+ "stations": "Gerst City,Clark City,Levinson Penal colony"
+ },
+ {
+ "name": "HIP 19808",
+ "pos_x": 1.53125,
+ "pos_y": -53.15625,
+ "pos_z": -110.0625,
+ "stations": "King Hub,Lasswitz Dock,Bethke Beacon,Jenkinson Installation"
+ },
+ {
+ "name": "HIP 19809",
+ "pos_x": 46.21875,
+ "pos_y": -107.8125,
+ "pos_z": -138.53125,
+ "stations": "Atwater Orbital,Newton Hub,Burton Base"
+ },
+ {
+ "name": "HIP 19869",
+ "pos_x": 177.3125,
+ "pos_y": -168.25,
+ "pos_z": -11.09375,
+ "stations": "McMillan Barracks,Elmore's Pride,Palisa Colony,Hawker Installation"
+ },
+ {
+ "name": "HIP 1987",
+ "pos_x": -121.25,
+ "pos_y": -35.46875,
+ "pos_z": -66.90625,
+ "stations": "Lovell Station,Sharipov Beacon"
+ },
+ {
+ "name": "HIP 19875",
+ "pos_x": 106.9375,
+ "pos_y": -114.59375,
+ "pos_z": -34.96875,
+ "stations": "Ziewe Survey,Flammarion Hub"
+ },
+ {
+ "name": "HIP 19894",
+ "pos_x": 130.03125,
+ "pos_y": -159.0625,
+ "pos_z": -85.8125,
+ "stations": "Roed Odegaard Colony,Hayashi Terminal"
+ },
+ {
+ "name": "HIP 19912",
+ "pos_x": 9.46875,
+ "pos_y": -60.03125,
+ "pos_z": -111.5625,
+ "stations": "Reisman Point,Hovell Asylum"
+ },
+ {
+ "name": "HIP 19925",
+ "pos_x": 26.75,
+ "pos_y": -74.84375,
+ "pos_z": -109.625,
+ "stations": "J. G. Ballard Market,Fife Port,Nikitin Colony,Galindo Orbital,Moore Port,Wright Enterprise,Grassmann Orbital,Effinger Orbital,Bellamy Dock,Mitropoulos' Progress,Schmidt Terminal,Longyear's Inheritance,Nicholson Works"
+ },
+ {
+ "name": "HIP 19934",
+ "pos_x": -17.34375,
+ "pos_y": -57.59375,
+ "pos_z": -160.125,
+ "stations": "Fozard Dock"
+ },
+ {
+ "name": "HIP 19950",
+ "pos_x": 98.5625,
+ "pos_y": -135.71875,
+ "pos_z": -99.40625,
+ "stations": "Wright Hub,Hawkes Bastion"
+ },
+ {
+ "name": "HIP 2",
+ "pos_x": -33.78125,
+ "pos_y": -152,
+ "pos_z": 15.34375,
+ "stations": "Johri Settlement,Macgregor's Exile"
+ },
+ {
+ "name": "HIP 20002",
+ "pos_x": 97.1875,
+ "pos_y": -115.34375,
+ "pos_z": -58.71875,
+ "stations": "Ban Settlement,Wright Station,Comper Holdings"
+ },
+ {
+ "name": "HIP 20009",
+ "pos_x": 163.4375,
+ "pos_y": -173.46875,
+ "pos_z": -53.75,
+ "stations": "Hoffmeister's Claim,Vinge Relay"
+ },
+ {
+ "name": "HIP 20019",
+ "pos_x": -4.8125,
+ "pos_y": -57.78125,
+ "pos_z": -137.65625,
+ "stations": "Selye Orbital,Forsskal Depot,Dunbar Gateway,Collins Laboratory"
+ },
+ {
+ "name": "HIP 20024",
+ "pos_x": 131.40625,
+ "pos_y": -157.34375,
+ "pos_z": -83.34375,
+ "stations": "Shen Reformatory,Roelofs Horizons"
+ },
+ {
+ "name": "HIP 20048",
+ "pos_x": 78.25,
+ "pos_y": -90.625,
+ "pos_z": -43.15625,
+ "stations": "Hooker Orbital,Rosseland Hub,Williams Silo,Mattei Station"
+ },
+ {
+ "name": "HIP 20052",
+ "pos_x": 123.0625,
+ "pos_y": -130.59375,
+ "pos_z": -41.46875,
+ "stations": "Rubin Hub,Ambartsumian Station"
+ },
+ {
+ "name": "HIP 20056",
+ "pos_x": -7.375,
+ "pos_y": -56.34375,
+ "pos_z": -140.4375,
+ "stations": "Boole Installation,Low Prospect,Markham Landing"
+ },
+ {
+ "name": "HIP 20084",
+ "pos_x": -10.9375,
+ "pos_y": -50.125,
+ "pos_z": -134.375,
+ "stations": "Zillig Port,Womack Beacon"
+ },
+ {
+ "name": "HIP 20093",
+ "pos_x": 43.25,
+ "pos_y": -81.4375,
+ "pos_z": -94.53125,
+ "stations": "Bulleid Enterprise,Grissom Observatory"
+ },
+ {
+ "name": "HIP 20094",
+ "pos_x": -39.15625,
+ "pos_y": -23.59375,
+ "pos_z": -132.59375,
+ "stations": "Lucid Horizons,White Orbital,Borel Arsenal,Parazynski Vision"
+ },
+ {
+ "name": "HIP 20109",
+ "pos_x": 167.3125,
+ "pos_y": -163.0625,
+ "pos_z": -25.40625,
+ "stations": "Xuesen Prospect,Wetherill Port"
+ },
+ {
+ "name": "HIP 20169",
+ "pos_x": 19.25,
+ "pos_y": -88.71875,
+ "pos_z": -161.96875,
+ "stations": "Sterling Hub"
+ },
+ {
+ "name": "HIP 20184",
+ "pos_x": 97.71875,
+ "pos_y": -127.28125,
+ "pos_z": -88.9375,
+ "stations": "Pritchard Orbital,Al Sufi Terminal,De Lay Mines,Kulin Plant"
+ },
+ {
+ "name": "HIP 20199",
+ "pos_x": 95.84375,
+ "pos_y": -105.0625,
+ "pos_z": -42.40625,
+ "stations": "Fabricius Vision,Elson Terminal"
+ },
+ {
+ "name": "HIP 20218",
+ "pos_x": 31.90625,
+ "pos_y": -71.0625,
+ "pos_z": -96.875,
+ "stations": "Gardner Station"
+ },
+ {
+ "name": "HIP 20237",
+ "pos_x": -7.875,
+ "pos_y": -49.96875,
+ "pos_z": -131.25,
+ "stations": "Padalka Market"
+ },
+ {
+ "name": "HIP 20277",
+ "pos_x": 106.4375,
+ "pos_y": -95.6875,
+ "pos_z": -0.1875,
+ "stations": "Fabian City,Trujillo Prospect,Lemonnier Survey"
+ },
+ {
+ "name": "HIP 20342",
+ "pos_x": 57.375,
+ "pos_y": -83.25,
+ "pos_z": -74.78125,
+ "stations": "Bowen City,Gordon Barracks"
+ },
+ {
+ "name": "HIP 20347",
+ "pos_x": 97.15625,
+ "pos_y": -130.25,
+ "pos_z": -101.59375,
+ "stations": "Mies van der Rohe Beacon"
+ },
+ {
+ "name": "HIP 20350",
+ "pos_x": -6.78125,
+ "pos_y": -58.53125,
+ "pos_z": -151.5625,
+ "stations": "Bond Beacon,Lee Point"
+ },
+ {
+ "name": "HIP 20385",
+ "pos_x": 156.96875,
+ "pos_y": -171.125,
+ "pos_z": -72.9375,
+ "stations": "Chernykh Legacy,Boss Vision"
+ },
+ {
+ "name": "HIP 20395",
+ "pos_x": -57.03125,
+ "pos_y": -19.9375,
+ "pos_z": -167.40625,
+ "stations": "Crown Station"
+ },
+ {
+ "name": "HIP 20410",
+ "pos_x": 28.96875,
+ "pos_y": -96.0625,
+ "pos_z": -166.5,
+ "stations": "Katzenstein Base,Gerrold Base,McCarthy Relay"
+ },
+ {
+ "name": "HIP 20419",
+ "pos_x": 9.625,
+ "pos_y": -73.0625,
+ "pos_z": -153.375,
+ "stations": "Tull Vision,Geston Point"
+ },
+ {
+ "name": "HIP 20440",
+ "pos_x": 0.96875,
+ "pos_y": -55.6875,
+ "pos_z": -130.96875,
+ "stations": "Wright Gateway,McMullen's Folly,Gerlache Exchange"
+ },
+ {
+ "name": "HIP 20441",
+ "pos_x": -2.125,
+ "pos_y": -48.125,
+ "pos_z": -119.53125,
+ "stations": "Culbertson Port,Miller Horizons,Katzenstein Installation,Brand Dock"
+ },
+ {
+ "name": "HIP 20478",
+ "pos_x": 70.9375,
+ "pos_y": -94.0625,
+ "pos_z": -74.96875,
+ "stations": "Curtiss Base,Neville Installation"
+ },
+ {
+ "name": "HIP 20480",
+ "pos_x": -12.5625,
+ "pos_y": -53,
+ "pos_z": -154.78125,
+ "stations": "von Krusenstern Terminal"
+ },
+ {
+ "name": "HIP 20485",
+ "pos_x": -0.125,
+ "pos_y": -58.625,
+ "pos_z": -141.8125,
+ "stations": "Shinn Enterprise,Bachman Port,Macedo Hub,Vlamingh's Claim,Fancher Orbital,Kazantsev Terminal,Barnaby Enterprise"
+ },
+ {
+ "name": "HIP 20491",
+ "pos_x": -19.40625,
+ "pos_y": -48.0625,
+ "pos_z": -157.8125,
+ "stations": "Nourse Depot,Semeonis Survey"
+ },
+ {
+ "name": "HIP 20492",
+ "pos_x": 2.15625,
+ "pos_y": -59.5,
+ "pos_z": -139.3125,
+ "stations": "Zajdel City,Moresby Station,Barr Barracks"
+ },
+ {
+ "name": "HIP 20509",
+ "pos_x": 112.84375,
+ "pos_y": -141.96875,
+ "pos_z": -101.90625,
+ "stations": "Riccioli Landing,Payne-Gaposchkin Colony,Sitter Silo"
+ },
+ {
+ "name": "HIP 20524",
+ "pos_x": 93.5,
+ "pos_y": -108.625,
+ "pos_z": -62.875,
+ "stations": "Marcy Enterprise,Young Terminal,Caidin Relay"
+ },
+ {
+ "name": "HIP 20527",
+ "pos_x": 3.625,
+ "pos_y": -61.15625,
+ "pos_z": -141,
+ "stations": "Wundt Ring,Blenkinsop Barracks,Merbold Terminal,Kanwar Terminal,Krylov Horizons"
+ },
+ {
+ "name": "HIP 20528",
+ "pos_x": -27,
+ "pos_y": -41.8125,
+ "pos_z": -159.875,
+ "stations": "Hoshi's Claim,Silva Survey,Tanner's Folly"
+ },
+ {
+ "name": "HIP 20541",
+ "pos_x": -99.1875,
+ "pos_y": 12.09375,
+ "pos_z": -184.4375,
+ "stations": "Byrd Installation"
+ },
+ {
+ "name": "HIP 20551",
+ "pos_x": 141.84375,
+ "pos_y": -142.71875,
+ "pos_z": -42.4375,
+ "stations": "Sandage Settlement,Mueller Dock,Hutchinson Enterprise"
+ },
+ {
+ "name": "HIP 20561",
+ "pos_x": 138.5,
+ "pos_y": -140.09375,
+ "pos_z": -43.3125,
+ "stations": "Meuron Landing,Thomson Survey,Brunner Vision"
+ },
+ {
+ "name": "HIP 20577",
+ "pos_x": -2.0625,
+ "pos_y": -57.5625,
+ "pos_z": -145.78125,
+ "stations": "Morgan Relay,du Fresne Works,Busch Settlement"
+ },
+ {
+ "name": "HIP 20586",
+ "pos_x": -67.4375,
+ "pos_y": 4,
+ "pos_z": -136.3125,
+ "stations": "Haxel Silo,Lukyanenko Enterprise"
+ },
+ {
+ "name": "HIP 20594",
+ "pos_x": 116.46875,
+ "pos_y": -135.28125,
+ "pos_z": -80.125,
+ "stations": "Gorgani Outpost,Hoffmeister Beacon"
+ },
+ {
+ "name": "HIP 20606",
+ "pos_x": 126.75,
+ "pos_y": -122.53125,
+ "pos_z": -26.78125,
+ "stations": "Whitford Terminal"
+ },
+ {
+ "name": "HIP 20610",
+ "pos_x": 162.84375,
+ "pos_y": -118.21875,
+ "pos_z": 62.40625,
+ "stations": "Charlier City,Dickson Landing"
+ },
+ {
+ "name": "HIP 20611",
+ "pos_x": 155.40625,
+ "pos_y": -159.84375,
+ "pos_z": -56.65625,
+ "stations": "Hoffleit's Exile"
+ },
+ {
+ "name": "HIP 20616",
+ "pos_x": 8.625,
+ "pos_y": -65.59375,
+ "pos_z": -143.53125,
+ "stations": "Normand Hub,Crown's Progress"
+ },
+ {
+ "name": "HIP 20638",
+ "pos_x": 85.90625,
+ "pos_y": -97.59375,
+ "pos_z": -54.9375,
+ "stations": "Islam Works"
+ },
+ {
+ "name": "HIP 20673",
+ "pos_x": 34.0625,
+ "pos_y": -74.90625,
+ "pos_z": -112.53125,
+ "stations": "Russo Vision"
+ },
+ {
+ "name": "HIP 20679",
+ "pos_x": -3.9375,
+ "pos_y": -55.15625,
+ "pos_z": -146.84375,
+ "stations": "Goonan Orbital,Hugh Terminal"
+ },
+ {
+ "name": "HIP 20686",
+ "pos_x": -5.25,
+ "pos_y": -49.46875,
+ "pos_z": -135.625,
+ "stations": "Hawley Terminal,Butcher Point,Hoshi Lab"
+ },
+ {
+ "name": "HIP 20719",
+ "pos_x": -1.125,
+ "pos_y": -53.15625,
+ "pos_z": -136.90625,
+ "stations": "Jacquard Horizons,Stebler Hangar,Samuda's Folly"
+ },
+ {
+ "name": "HIP 20722",
+ "pos_x": -62.03125,
+ "pos_y": 6.78125,
+ "pos_z": -120.125,
+ "stations": "Richards Vision,Bradley Depot"
+ },
+ {
+ "name": "HIP 20723",
+ "pos_x": 48.375,
+ "pos_y": -81.875,
+ "pos_z": -100.15625,
+ "stations": "Parsons Landing,Woodroffe Horizons"
+ },
+ {
+ "name": "HIP 20727",
+ "pos_x": 104.78125,
+ "pos_y": -122.1875,
+ "pos_z": -77.46875,
+ "stations": "Hatanaka Port,Vess Station,Stokes Hub"
+ },
+ {
+ "name": "HIP 20737",
+ "pos_x": 67.96875,
+ "pos_y": -83.21875,
+ "pos_z": -60.46875,
+ "stations": "Lintott City,Ruppelt Vision,Verne Hub,Leonard Legacy,Okorafor Prospect,Leopold Heckmann Ring,Korlevic Port,Bacigalupi Prospect,Chern Vision,Kurland's Inheritance"
+ },
+ {
+ "name": "HIP 20741",
+ "pos_x": -0.84375,
+ "pos_y": -54.375,
+ "pos_z": -139.9375,
+ "stations": "Teng Dock"
+ },
+ {
+ "name": "HIP 20762",
+ "pos_x": 6.3125,
+ "pos_y": -57.09375,
+ "pos_z": -131.53125,
+ "stations": "Tiedemann Settlement,Siodmak Plant,Blair Camp"
+ },
+ {
+ "name": "HIP 20777",
+ "pos_x": -15.90625,
+ "pos_y": -33.59375,
+ "pos_z": -121.28125,
+ "stations": "Landsteiner Terminal,Chadwick Ring,Crouch Dock,Matheson Settlement"
+ },
+ {
+ "name": "HIP 20792",
+ "pos_x": 163.53125,
+ "pos_y": -158.125,
+ "pos_z": -40.1875,
+ "stations": "Rankin Terminal,Zubrin Vision,Gehry Station"
+ },
+ {
+ "name": "HIP 20806",
+ "pos_x": 69.59375,
+ "pos_y": -101.4375,
+ "pos_z": -105.25,
+ "stations": "Parker Vision,Addams Terminal,Ashman Platform"
+ },
+ {
+ "name": "HIP 20826",
+ "pos_x": 9.875,
+ "pos_y": -61.9375,
+ "pos_z": -137.90625,
+ "stations": "Murphy Dock,Tavernier Landing,Thesiger Installation,Almagro Dock,Sabine Hub"
+ },
+ {
+ "name": "HIP 20827",
+ "pos_x": 4.96875,
+ "pos_y": -65.65625,
+ "pos_z": -158.5625,
+ "stations": "Clerk Depot,Still Port,Birkeland Installation"
+ },
+ {
+ "name": "HIP 20850",
+ "pos_x": 5.53125,
+ "pos_y": -57.75,
+ "pos_z": -137.5,
+ "stations": "White's Folly,Samuda's Claim"
+ },
+ {
+ "name": "HIP 20869",
+ "pos_x": 211.3125,
+ "pos_y": -172.78125,
+ "pos_z": 27.25,
+ "stations": "Asami Port,Jung Landing,Riccioli Mines"
+ },
+ {
+ "name": "HIP 20890",
+ "pos_x": -6.3125,
+ "pos_y": -50.125,
+ "pos_z": -145.71875,
+ "stations": "Asher Barracks,Foden Landing"
+ },
+ {
+ "name": "HIP 20895",
+ "pos_x": -15.5,
+ "pos_y": -30.75,
+ "pos_z": -115.8125,
+ "stations": "Rayhan al-Biruni Relay,MacKellar Station"
+ },
+ {
+ "name": "HIP 20899",
+ "pos_x": -1.09375,
+ "pos_y": -53.09375,
+ "pos_z": -142.0625,
+ "stations": "Maury Landing,Morgan Bastion"
+ },
+ {
+ "name": "HIP 20916",
+ "pos_x": 1.34375,
+ "pos_y": -57.03125,
+ "pos_z": -147.3125,
+ "stations": "Davidson Platform,Weil Terminal,Dozois Port,Grothendieck Colony"
+ },
+ {
+ "name": "HIP 20920",
+ "pos_x": 205.5,
+ "pos_y": -161.15625,
+ "pos_z": 43.34375,
+ "stations": "Stone Station,Hiyya Prospect"
+ },
+ {
+ "name": "HIP 20924",
+ "pos_x": 172.78125,
+ "pos_y": -162.40625,
+ "pos_z": -34.71875,
+ "stations": "Dornier Landing,Cartier Hub,Banks Installation"
+ },
+ {
+ "name": "HIP 20935",
+ "pos_x": -1.3125,
+ "pos_y": -48.59375,
+ "pos_z": -131.875,
+ "stations": "Kizim Dock,McKay Terminal,Kanwar Port,Morukov Vision"
+ },
+ {
+ "name": "HIP 20948",
+ "pos_x": -2,
+ "pos_y": -51.78125,
+ "pos_z": -142.21875,
+ "stations": "Fernao do Po Base"
+ },
+ {
+ "name": "HIP 20968",
+ "pos_x": 71.125,
+ "pos_y": -84.71875,
+ "pos_z": -62.625,
+ "stations": "Homer Landing"
+ },
+ {
+ "name": "HIP 20978",
+ "pos_x": 0.53125,
+ "pos_y": -47,
+ "pos_z": -124.59375,
+ "stations": "Humboldt City,Avogadro Holdings,Nelson Point"
+ },
+ {
+ "name": "HIP 20998",
+ "pos_x": 130.71875,
+ "pos_y": -103.96875,
+ "pos_z": 22.65625,
+ "stations": "Louis de Lacaille Orbital,van Rhijn Vista,Bryant Point"
+ },
+ {
+ "name": "HIP 21010",
+ "pos_x": -18.84375,
+ "pos_y": -28.75,
+ "pos_z": -121.03125,
+ "stations": "Bates Barracks,Lupoff Plant,Garnier Orbital"
+ },
+ {
+ "name": "HIP 2102",
+ "pos_x": -104.4375,
+ "pos_y": -73.875,
+ "pos_z": -53.90625,
+ "stations": "Mallory Orbital,Bao Keep"
+ },
+ {
+ "name": "HIP 21053",
+ "pos_x": 1.8125,
+ "pos_y": -48.1875,
+ "pos_z": -126.53125,
+ "stations": "Ross Terminal,Kuttner Hub,Griffiths Hub,Abbott Orbital,Lambert Lab"
+ },
+ {
+ "name": "HIP 21066",
+ "pos_x": 12.6875,
+ "pos_y": -59.5625,
+ "pos_z": -132.5,
+ "stations": "Bulgakov Hub,Sterling Station,Balmer Horizons,Nixon Arsenal,Lander Vision"
+ },
+ {
+ "name": "HIP 21069",
+ "pos_x": -41.5625,
+ "pos_y": -23.8125,
+ "pos_z": -161.71875,
+ "stations": "Tuan Bastion,Pond Works"
+ },
+ {
+ "name": "HIP 21112",
+ "pos_x": 7.8125,
+ "pos_y": -64.21875,
+ "pos_z": -158.0625,
+ "stations": "Conklin Port,Killough Base,Harness Enterprise,Felice Station,Bester Vision"
+ },
+ {
+ "name": "HIP 21123",
+ "pos_x": -0.8125,
+ "pos_y": -48.34375,
+ "pos_z": -134.9375,
+ "stations": "Reynolds Bastion,Farkas Port,Barry Base"
+ },
+ {
+ "name": "HIP 21138",
+ "pos_x": 4.28125,
+ "pos_y": -61.25,
+ "pos_z": -159.1875,
+ "stations": "Fermat Colony,Holland Survey"
+ },
+ {
+ "name": "HIP 21143",
+ "pos_x": 70.6875,
+ "pos_y": -106.6875,
+ "pos_z": -128.4375,
+ "stations": "Samuda Lab"
+ },
+ {
+ "name": "HIP 21165",
+ "pos_x": -94.15625,
+ "pos_y": 47.625,
+ "pos_z": -90.5625,
+ "stations": "Kidman Orbital,Bischoff Landing,Perez Terminal,Morris Barracks"
+ },
+ {
+ "name": "HIP 21167",
+ "pos_x": -37.21875,
+ "pos_y": -17.1875,
+ "pos_z": -136.03125,
+ "stations": "Levi-Civita Dock,Moore Depot,Magnus Holdings,Barth Mine"
+ },
+ {
+ "name": "HIP 21179",
+ "pos_x": 10.84375,
+ "pos_y": -70,
+ "pos_z": -169.5625,
+ "stations": "Samos Works,Nielsen Terminal"
+ },
+ {
+ "name": "HIP 21187",
+ "pos_x": 150,
+ "pos_y": -132.03125,
+ "pos_z": -12.46875,
+ "stations": "Kepler Survey,Herndon Enterprise,Peters Station,Leydenfrost Refinery"
+ },
+ {
+ "name": "HIP 21256",
+ "pos_x": -5.78125,
+ "pos_y": -36,
+ "pos_z": -116.40625,
+ "stations": "Snyder Works"
+ },
+ {
+ "name": "HIP 21261",
+ "pos_x": -2.8125,
+ "pos_y": -49.5625,
+ "pos_z": -148.0625,
+ "stations": "Scortia Camp,Clement Platform"
+ },
+ {
+ "name": "HIP 21267",
+ "pos_x": 8.84375,
+ "pos_y": -54.6875,
+ "pos_z": -134.75,
+ "stations": "Sternberg Platform,Riemann Laboratory"
+ },
+ {
+ "name": "HIP 21280",
+ "pos_x": 4.78125,
+ "pos_y": -48.3125,
+ "pos_z": -126.84375,
+ "stations": "Lethem Barracks,Zamka Dock,Brunton Dock,Singer Enterprise,Boswell Hub,Buchli Orbital,Kippax Beacon,Shepard Terminal,Zalyotin City,Walker Lab"
+ },
+ {
+ "name": "HIP 21286",
+ "pos_x": 145.78125,
+ "pos_y": -123.34375,
+ "pos_z": -0.53125,
+ "stations": "Hansen Point,Dayuan Prospect"
+ },
+ {
+ "name": "HIP 21308",
+ "pos_x": 17.4375,
+ "pos_y": -66.125,
+ "pos_z": -148.625,
+ "stations": "Preuss Relay"
+ },
+ {
+ "name": "HIP 2136",
+ "pos_x": 15.75,
+ "pos_y": -166.3125,
+ "pos_z": 25.65625,
+ "stations": "Oshima Estate"
+ },
+ {
+ "name": "HIP 21369",
+ "pos_x": -94.5,
+ "pos_y": 23.5625,
+ "pos_z": -163.125,
+ "stations": "Biggle Escape"
+ },
+ {
+ "name": "HIP 21380",
+ "pos_x": -10.71875,
+ "pos_y": -42,
+ "pos_z": -149.40625,
+ "stations": "Beaufoy Port,Caryanda Legacy,Lukyanenko Survey"
+ },
+ {
+ "name": "HIP 21383",
+ "pos_x": 38.5625,
+ "pos_y": -66.96875,
+ "pos_z": -101.375,
+ "stations": "Adkins Port,Barlowe Orbital"
+ },
+ {
+ "name": "HIP 21414",
+ "pos_x": 89.84375,
+ "pos_y": -112.28125,
+ "pos_z": -109.28125,
+ "stations": "Madsen Port,Furukawa Prospect,Fedden Relay"
+ },
+ {
+ "name": "HIP 21431",
+ "pos_x": 137.75,
+ "pos_y": -131.78125,
+ "pos_z": -49.03125,
+ "stations": "Palmer Silo,Whittle Settlement,Seki Hub"
+ },
+ {
+ "name": "HIP 21438",
+ "pos_x": 122.59375,
+ "pos_y": -123.75,
+ "pos_z": -63.25,
+ "stations": "Melia Gateway,Cook Silo"
+ },
+ {
+ "name": "HIP 21455",
+ "pos_x": 109.90625,
+ "pos_y": -74.09375,
+ "pos_z": 52.96875,
+ "stations": "Barnaby Gateway,Kier Enterprise,Crook Terminal"
+ },
+ {
+ "name": "HIP 21458",
+ "pos_x": 145.71875,
+ "pos_y": -137.375,
+ "pos_z": -46.75,
+ "stations": "Cummings Mines,Foglio Vision"
+ },
+ {
+ "name": "HIP 21459",
+ "pos_x": -10.84375,
+ "pos_y": -40.8125,
+ "pos_z": -149.1875,
+ "stations": "Daniel Landing,MacVicar Colony,Abbott Keep,Waldrop Enterprise"
+ },
+ {
+ "name": "HIP 21474",
+ "pos_x": 4.84375,
+ "pos_y": -50.6875,
+ "pos_z": -139.96875,
+ "stations": "Stanier Survey,Khan Settlement,Nelder Hub"
+ },
+ {
+ "name": "HIP 21559",
+ "pos_x": -42.09375,
+ "pos_y": -7.40625,
+ "pos_z": -129.5,
+ "stations": "Roberts Horizons,Morukov Terminal,Harris Enterprise,Burbank Port,Lenoir City,Born City,Khayyam Hub,Lorentz Dock,Gibson's Inheritance,Georg Bothe Gateway,Woolley Hub,Georg Bothe Enterprise,Mendeleev's Folly,Zholobov's Progress,Flynn Horizons"
+ },
+ {
+ "name": "HIP 21609",
+ "pos_x": 147.53125,
+ "pos_y": -116.6875,
+ "pos_z": 16.9375,
+ "stations": "Adragna Station,Smith Vista"
+ },
+ {
+ "name": "HIP 21632",
+ "pos_x": 103,
+ "pos_y": -116.34375,
+ "pos_z": -97.125,
+ "stations": "Flint Penal colony,Laumer Vision,Moffitt Port,Drummond Landing,Vonarburg Terminal"
+ },
+ {
+ "name": "HIP 21637",
+ "pos_x": -8.9375,
+ "pos_y": -37.5,
+ "pos_z": -140.03125,
+ "stations": "Ordway Survey"
+ },
+ {
+ "name": "HIP 21723",
+ "pos_x": 11.71875,
+ "pos_y": -50.5,
+ "pos_z": -129.84375,
+ "stations": "Windt Hub,Martin Enterprise,Langsdorff Station,Sagan Depot"
+ },
+ {
+ "name": "HIP 21724",
+ "pos_x": 149.4375,
+ "pos_y": -152.5,
+ "pos_z": -94.09375,
+ "stations": "Antonov Hub,Baynes Prospect,Addams Station,Dalgarno Settlement,Due Beacon"
+ },
+ {
+ "name": "HIP 21750",
+ "pos_x": -82.875,
+ "pos_y": 34.34375,
+ "pos_z": -107.71875,
+ "stations": "McCormick Dock,Sarafanov Survey"
+ },
+ {
+ "name": "HIP 2177",
+ "pos_x": 5.46875,
+ "pos_y": -238.84375,
+ "pos_z": 26.375,
+ "stations": "Piercy Landing,McKean Platform"
+ },
+ {
+ "name": "HIP 21778",
+ "pos_x": 79.90625,
+ "pos_y": -87.53125,
+ "pos_z": -71.25,
+ "stations": "Gibson Port,Crown Gateway,Lundwall Hub,Barreiros Orbital,Ehrenfried Kegel Dock,Serebrov Arsenal,Cartmill Orbital,Vogel Plant,Coelho City,Carlisle City,Fan Installation,Warner Dock,Davis Base,Gora's Progress,Carr Settlement"
+ },
+ {
+ "name": "HIP 21821",
+ "pos_x": 138,
+ "pos_y": -119.96875,
+ "pos_z": -23.6875,
+ "stations": "Kawasato Gateway,Lee Dock,Michael Holdings,Slade Base,Cremona Horizons,Biesbroeck Station"
+ },
+ {
+ "name": "HIP 21879",
+ "pos_x": 133.75,
+ "pos_y": -118.875,
+ "pos_z": -33.375,
+ "stations": "Kobayashi Hangar,Fehrenbach Refinery,Kopal Vision,Bergerac Silo"
+ },
+ {
+ "name": "HIP 21888",
+ "pos_x": 165.78125,
+ "pos_y": -153.59375,
+ "pos_z": -62.5,
+ "stations": "Parker Enterprise,Kohoutek Station,Kaluta Terminal,Koontz Base,Helffrich Terminal"
+ },
+ {
+ "name": "HIP 21912",
+ "pos_x": 6.9375,
+ "pos_y": -55.25,
+ "pos_z": -166.40625,
+ "stations": "Spinrad Horizons,Cantor Horizons,Murphy Landing"
+ },
+ {
+ "name": "HIP 21918",
+ "pos_x": 18.0625,
+ "pos_y": -65.28125,
+ "pos_z": -170.0625,
+ "stations": "Obruchev Port,Houtman Landing,MacDonald Horizons"
+ },
+ {
+ "name": "HIP 21923",
+ "pos_x": 1.34375,
+ "pos_y": -41.4375,
+ "pos_z": -135.6875,
+ "stations": "Samuda Enterprise"
+ },
+ {
+ "name": "HIP 21946",
+ "pos_x": 1.53125,
+ "pos_y": -45.09375,
+ "pos_z": -147.875,
+ "stations": "Young Stop,Toll Laboratory"
+ },
+ {
+ "name": "HIP 21991",
+ "pos_x": 73,
+ "pos_y": -91.03125,
+ "pos_z": -109.09375,
+ "stations": "Charlois City,Maler Base"
+ },
+ {
+ "name": "HIP 22006",
+ "pos_x": 100.5625,
+ "pos_y": -89.34375,
+ "pos_z": -27.75,
+ "stations": "Joseph Delambre Hub"
+ },
+ {
+ "name": "HIP 2201",
+ "pos_x": -53,
+ "pos_y": -141.8125,
+ "pos_z": -17.78125,
+ "stations": "Torricelli Colony"
+ },
+ {
+ "name": "HIP 22052",
+ "pos_x": 70.15625,
+ "pos_y": -86.21875,
+ "pos_z": -103.28125,
+ "stations": "Cavendish Colony,Gregory Hub,Al-Khalili's Progress"
+ },
+ {
+ "name": "HIP 22145",
+ "pos_x": 183.03125,
+ "pos_y": -139.625,
+ "pos_z": 24.34375,
+ "stations": "Bell Point,Baliunas Mines"
+ },
+ {
+ "name": "HIP 22150",
+ "pos_x": 220.875,
+ "pos_y": -169.03125,
+ "pos_z": 27.3125,
+ "stations": "Webb Beacon,Okuni Beacon,Baldwin Keep"
+ },
+ {
+ "name": "HIP 22177",
+ "pos_x": 31.65625,
+ "pos_y": -59.84375,
+ "pos_z": -124.28125,
+ "stations": "Lefschetz Legacy"
+ },
+ {
+ "name": "HIP 22192",
+ "pos_x": 108.15625,
+ "pos_y": -112.875,
+ "pos_z": -95.59375,
+ "stations": "Curtis Terminal,Hanke-Woods Survey,Moon Laboratory"
+ },
+ {
+ "name": "HIP 22221",
+ "pos_x": 19.8125,
+ "pos_y": -48.53125,
+ "pos_z": -118.75,
+ "stations": "Bosch Dock"
+ },
+ {
+ "name": "HIP 22224",
+ "pos_x": 4.4375,
+ "pos_y": -39.1875,
+ "pos_z": -129.53125,
+ "stations": "Brunel Colony,Brust's Folly,Adams Base,Brahe Settlement"
+ },
+ {
+ "name": "HIP 22229",
+ "pos_x": 158.84375,
+ "pos_y": -126.3125,
+ "pos_z": 0.84375,
+ "stations": "Alvarez de Pineda Settlement,Dominique Port,Rigaux Survey"
+ },
+ {
+ "name": "HIP 22249",
+ "pos_x": 175.875,
+ "pos_y": -138.65625,
+ "pos_z": 4.21875,
+ "stations": "Denning Landing,Verrazzano Penitentiary,Bentham Relay"
+ },
+ {
+ "name": "HIP 22271",
+ "pos_x": -11.75,
+ "pos_y": -28.90625,
+ "pos_z": -141.4375,
+ "stations": "Oswald Ring,Sinisalo Installation,Gaultier de Varennes Landing"
+ },
+ {
+ "name": "HIP 22278",
+ "pos_x": 176.0625,
+ "pos_y": -141.78125,
+ "pos_z": -7.8125,
+ "stations": "Seitter Prospect"
+ },
+ {
+ "name": "HIP 22281",
+ "pos_x": 84.15625,
+ "pos_y": -77.6875,
+ "pos_z": -40.53125,
+ "stations": "King Point,Lockwood Station,Hughes Port,Curtis Terminal,Condit Orbital,Rosse Gateway,Chelomey Vision,Lomonosov Vision,Libeskind Station,Lee Orbital,Regiomontanus Forum,Bullialdus Beacon,Foden Forum,Thompson Laboratory,Bellamy Holdings"
+ },
+ {
+ "name": "HIP 22286",
+ "pos_x": 115.90625,
+ "pos_y": -105.65625,
+ "pos_z": -51.21875,
+ "stations": "Tousey Vision,Allen Vista"
+ },
+ {
+ "name": "HIP 22295",
+ "pos_x": 155.0625,
+ "pos_y": -104.65625,
+ "pos_z": 67.84375,
+ "stations": "van de Sande Bakhuyzen Platform,Brorsen Landing"
+ },
+ {
+ "name": "HIP 22296",
+ "pos_x": 46.28125,
+ "pos_y": -76.40625,
+ "pos_z": -148.21875,
+ "stations": "Butler Orbital,Russo Mines,Remek Mines"
+ },
+ {
+ "name": "HIP 223",
+ "pos_x": -71.5625,
+ "pos_y": -118.5,
+ "pos_z": -13.28125,
+ "stations": "Ings Terminal,Gibson Ring,Heaviside City,Smith Beacon,Porges Depot"
+ },
+ {
+ "name": "HIP 22320",
+ "pos_x": 111.40625,
+ "pos_y": -113.8125,
+ "pos_z": -96.59375,
+ "stations": "Foster Orbital,Delaunay Dock,Belyavsky Hub,Anderson Installation"
+ },
+ {
+ "name": "HIP 22373",
+ "pos_x": 86.96875,
+ "pos_y": -88.21875,
+ "pos_z": -74.3125,
+ "stations": "Hampson Vision"
+ },
+ {
+ "name": "HIP 22380",
+ "pos_x": 3.8125,
+ "pos_y": -40.03125,
+ "pos_z": -140.78125,
+ "stations": "Redi Dock,Skvortsov Port"
+ },
+ {
+ "name": "HIP 22387",
+ "pos_x": 113.1875,
+ "pos_y": -108.21875,
+ "pos_z": -72.3125,
+ "stations": "Yuzhe Depot"
+ },
+ {
+ "name": "HIP 2239",
+ "pos_x": -2.46875,
+ "pos_y": -179.75,
+ "pos_z": 15.1875,
+ "stations": "Angstrom Hub,Kojima Port,Donaldson's Progress,Helffrich Orbital"
+ },
+ {
+ "name": "HIP 22394",
+ "pos_x": -9.5625,
+ "pos_y": -33.96875,
+ "pos_z": -158.3125,
+ "stations": "Grimwood Landing"
+ },
+ {
+ "name": "HIP 22395",
+ "pos_x": 83.5625,
+ "pos_y": -82.46875,
+ "pos_z": -63.34375,
+ "stations": "McKee Station,Fan Port,West Gateway,Minkowski Keep,Wafa's Folly,Cassini Observatory"
+ },
+ {
+ "name": "HIP 22496",
+ "pos_x": 8.03125,
+ "pos_y": -46.125,
+ "pos_z": -155.8125,
+ "stations": "Gagarin Terminal,Herbert Silo"
+ },
+ {
+ "name": "HIP 22500",
+ "pos_x": 121.6875,
+ "pos_y": -111.96875,
+ "pos_z": -64.9375,
+ "stations": "Mitra Orbital,Alexander Orbital,Lopez-Garcia Terminal"
+ },
+ {
+ "name": "HIP 22506",
+ "pos_x": 121.125,
+ "pos_y": -109.1875,
+ "pos_z": -56.21875,
+ "stations": "Goryu Hub,Lefschetz Keep,Hughes Plant"
+ },
+ {
+ "name": "HIP 22524",
+ "pos_x": 10.8125,
+ "pos_y": -49.15625,
+ "pos_z": -160.625,
+ "stations": "Liouville Prospect,Ivens' Claim"
+ },
+ {
+ "name": "HIP 22559",
+ "pos_x": -23.25,
+ "pos_y": -16.375,
+ "pos_z": -137.65625,
+ "stations": "Dobzhansky Gateway"
+ },
+ {
+ "name": "HIP 22566",
+ "pos_x": 14.28125,
+ "pos_y": -55.625,
+ "pos_z": -177.78125,
+ "stations": "Holden Horizons,Wilkes Hub,Viete Settlement,Plucker Horizons,Judson Base"
+ },
+ {
+ "name": "HIP 22607",
+ "pos_x": 13.5625,
+ "pos_y": -41.65625,
+ "pos_z": -125.40625,
+ "stations": "Harrison Beacon,Anthony de la Roche Point,Hand Base"
+ },
+ {
+ "name": "HIP 22611",
+ "pos_x": 126.90625,
+ "pos_y": -119.59375,
+ "pos_z": -84.03125,
+ "stations": "Gonnessiat Landing,Chadbourne Mines,Sinisalo Silo"
+ },
+ {
+ "name": "HIP 22615",
+ "pos_x": -76.03125,
+ "pos_y": 33.25,
+ "pos_z": -104.8125,
+ "stations": "Weston Horizons,Godwin Settlement,Pryor Point"
+ },
+ {
+ "name": "HIP 22616",
+ "pos_x": 25.09375,
+ "pos_y": -56.65625,
+ "pos_z": -150.1875,
+ "stations": "Phillifent Dock,Scortia Terminal,Abe Enterprise"
+ },
+ {
+ "name": "HIP 22654",
+ "pos_x": 4.46875,
+ "pos_y": -39.25,
+ "pos_z": -146.125,
+ "stations": "Pellegrino Landing,Wood Installation"
+ },
+ {
+ "name": "HIP 22703",
+ "pos_x": -126.1875,
+ "pos_y": 65.875,
+ "pos_z": -131.875,
+ "stations": "Filter's Claim,Selous' Progress"
+ },
+ {
+ "name": "HIP 2273",
+ "pos_x": -8.25,
+ "pos_y": -201.125,
+ "pos_z": 13.1875,
+ "stations": "Henry Port"
+ },
+ {
+ "name": "HIP 22771",
+ "pos_x": 112.625,
+ "pos_y": -105,
+ "pos_z": -76.46875,
+ "stations": "Bracewell Point,Bigourdan Laboratory"
+ },
+ {
+ "name": "HIP 22858",
+ "pos_x": -63.59375,
+ "pos_y": 6.59375,
+ "pos_z": -183.34375,
+ "stations": "Longyear Landing"
+ },
+ {
+ "name": "HIP 2293",
+ "pos_x": -142.96875,
+ "pos_y": 56,
+ "pos_z": -89.96875,
+ "stations": "Bloch Refinery"
+ },
+ {
+ "name": "HIP 22933",
+ "pos_x": 160.46875,
+ "pos_y": -119.53125,
+ "pos_z": 13.75,
+ "stations": "Hynek Lab,Kizawa Platform,Fukushima Vision,Watson Terminal"
+ },
+ {
+ "name": "HIP 22961",
+ "pos_x": -69.15625,
+ "pos_y": 29.8125,
+ "pos_z": -102.78125,
+ "stations": "Sternberg Survey,Saberhagen Orbital,Hudson Enterprise,Morrison Gateway,Marques' Folly"
+ },
+ {
+ "name": "HIP 23027",
+ "pos_x": -6,
+ "pos_y": -28.25,
+ "pos_z": -149.46875,
+ "stations": "Sawyer Vision,Corte-Real Dock,Sinisalo Hangar,Waldrop Hub,Chiang Terminal"
+ },
+ {
+ "name": "HIP 23042",
+ "pos_x": 134.03125,
+ "pos_y": -117.875,
+ "pos_z": -73.4375,
+ "stations": "Daqing Holdings,Oterma Landing,Hoffleit Prospect,Dahm Landing"
+ },
+ {
+ "name": "HIP 23046",
+ "pos_x": 177.8125,
+ "pos_y": -134.46875,
+ "pos_z": 2.59375,
+ "stations": "Greenstein Vision,Mattingly Point"
+ },
+ {
+ "name": "HIP 23100",
+ "pos_x": -39.375,
+ "pos_y": -3.15625,
+ "pos_z": -154.15625,
+ "stations": "King Port"
+ },
+ {
+ "name": "HIP 2311",
+ "pos_x": -44.3125,
+ "pos_y": -150.96875,
+ "pos_z": -13.0625,
+ "stations": "Cayley Vision,Arthur Silo,White Installation,Bradley Terminal,Balog Hub"
+ },
+ {
+ "name": "HIP 23155",
+ "pos_x": 24.65625,
+ "pos_y": -48.25,
+ "pos_z": -141.1875,
+ "stations": "Rochon Enterprise"
+ },
+ {
+ "name": "HIP 23157",
+ "pos_x": 156.71875,
+ "pos_y": -117.90625,
+ "pos_z": 1.5625,
+ "stations": "Tange Ring"
+ },
+ {
+ "name": "HIP 2318",
+ "pos_x": 18.90625,
+ "pos_y": -215.6875,
+ "pos_z": 30.1875,
+ "stations": "Russo Barracks,Lockyer Survey,Lagrange Prospect,Giunta Landing"
+ },
+ {
+ "name": "HIP 23185",
+ "pos_x": 153.59375,
+ "pos_y": -134.03125,
+ "pos_z": -88.375,
+ "stations": "Bolkow Dock,Fleming Platform,Lewis Ring"
+ },
+ {
+ "name": "HIP 2319",
+ "pos_x": 9.21875,
+ "pos_y": -242.5,
+ "pos_z": 26.75,
+ "stations": "Aller Station,Wenzel Orbital,Smyth Vision"
+ },
+ {
+ "name": "HIP 23248",
+ "pos_x": 84.59375,
+ "pos_y": -76.0625,
+ "pos_z": -61.9375,
+ "stations": "Goldreich Hub,Franklin Reach,Mead Dock,Woodroffe Forum,Skjellerup Orbital"
+ },
+ {
+ "name": "HIP 23259",
+ "pos_x": -4.65625,
+ "pos_y": -21.375,
+ "pos_z": -122.5625,
+ "stations": "Banks Port,Carr Colony,McMonagle Arsenal,Hudson Hub,Brunner Survey"
+ },
+ {
+ "name": "HIP 2327",
+ "pos_x": -106.625,
+ "pos_y": -107.9375,
+ "pos_z": -54.125,
+ "stations": "Eanes Arena,Chalker Mine"
+ },
+ {
+ "name": "HIP 23277",
+ "pos_x": 43.78125,
+ "pos_y": -65.75,
+ "pos_z": -163.3125,
+ "stations": "Peirce Port,Baird Orbital,Baraniecki Bastion,Nelson Landing"
+ },
+ {
+ "name": "HIP 23302",
+ "pos_x": -15.09375,
+ "pos_y": -14.25,
+ "pos_z": -127.15625,
+ "stations": "Galilei Port"
+ },
+ {
+ "name": "HIP 23317",
+ "pos_x": -76.03125,
+ "pos_y": 31.46875,
+ "pos_z": -126.96875,
+ "stations": "Tereshkova Ring,Linteris Hub,Meikle Landing,Berezin Silo"
+ },
+ {
+ "name": "HIP 23318",
+ "pos_x": -102.9375,
+ "pos_y": 56.625,
+ "pos_z": -101.8125,
+ "stations": "Hamilton Landing"
+ },
+ {
+ "name": "HIP 23395",
+ "pos_x": -7.34375,
+ "pos_y": -20.0625,
+ "pos_z": -130.71875,
+ "stations": "Dyomin Station,Hope Station,Jett Market,Gohar Port,Levchenko Dock"
+ },
+ {
+ "name": "HIP 23413",
+ "pos_x": 143.78125,
+ "pos_y": -97.53125,
+ "pos_z": 48.9375,
+ "stations": "Schwabe Platform,Barbuy Landing,Barbuy Hub,Nicollet Penal colony,Bolkow Landing"
+ },
+ {
+ "name": "HIP 23421",
+ "pos_x": 56.03125,
+ "pos_y": -74.28125,
+ "pos_z": -168.1875,
+ "stations": "Burstein Bastion,Carter Orbital,Salgari Dock"
+ },
+ {
+ "name": "HIP 23443",
+ "pos_x": 16.0625,
+ "pos_y": -35.46875,
+ "pos_z": -122.34375,
+ "stations": "Forstchen Hub,Carver Enterprise,Longyear Gateway,Linge Hub,Hambly Enterprise,Cole Enterprise,Ore Ring"
+ },
+ {
+ "name": "HIP 2350",
+ "pos_x": -57.46875,
+ "pos_y": -150.875,
+ "pos_z": -21.3125,
+ "stations": "Brahe Prospect,Popov Base"
+ },
+ {
+ "name": "HIP 23519",
+ "pos_x": 109.28125,
+ "pos_y": -100.28125,
+ "pos_z": -104.28125,
+ "stations": "Krylov Arena"
+ },
+ {
+ "name": "HIP 23575",
+ "pos_x": 54.21875,
+ "pos_y": -66.0625,
+ "pos_z": -142.5,
+ "stations": "Plucker Orbital,Kirk Laboratory,Salgari Colony,MacLeod Installation"
+ },
+ {
+ "name": "HIP 23627",
+ "pos_x": 62.78125,
+ "pos_y": -64.28125,
+ "pos_z": -101.15625,
+ "stations": "Bykovsky Enterprise"
+ },
+ {
+ "name": "HIP 2364",
+ "pos_x": -119.0625,
+ "pos_y": -19.46875,
+ "pos_z": -69.40625,
+ "stations": "McArthur Terminal"
+ },
+ {
+ "name": "HIP 23641",
+ "pos_x": 108,
+ "pos_y": -75.875,
+ "pos_z": 19.3125,
+ "stations": "Iben City,Szameit Depot,Weber Installation,Hussey Dock,Beg Ring,Iben Dock,Boscovich Gateway,Bliss Terminal,Michalitsianos Dock,Extra Station,Krigstein Terminal,Jenkinson Palace,Draper Point"
+ },
+ {
+ "name": "HIP 23655",
+ "pos_x": -49.625,
+ "pos_y": 5.8125,
+ "pos_z": -171.625,
+ "stations": "Pond Reach,Dorsey Mines"
+ },
+ {
+ "name": "HIP 23694",
+ "pos_x": -65.65625,
+ "pos_y": 21.78125,
+ "pos_z": -149.4375,
+ "stations": "Erdos Vision,Maddox Sanctuary"
+ },
+ {
+ "name": "HIP 23716",
+ "pos_x": 74.71875,
+ "pos_y": -73.71875,
+ "pos_z": -109.75,
+ "stations": "Knight Enterprise,Wakata Station,Wolf Orbital,Godel Escape,Farkas Oasis"
+ },
+ {
+ "name": "HIP 23719",
+ "pos_x": 167.84375,
+ "pos_y": -114.71875,
+ "pos_z": 45.78125,
+ "stations": "Edwards Port,Arnold Schwassmann Dock"
+ },
+ {
+ "name": "HIP 23759",
+ "pos_x": 359.84375,
+ "pos_y": -385.53125,
+ "pos_z": -718.375,
+ "stations": "Witch Head Science Centre"
+ },
+ {
+ "name": "HIP 23816",
+ "pos_x": 87.875,
+ "pos_y": -82.75,
+ "pos_z": -112.59375,
+ "stations": "Henson Dock,Khrenov Port,Robinson Terminal"
+ },
+ {
+ "name": "HIP 23818",
+ "pos_x": 68.40625,
+ "pos_y": -63.28125,
+ "pos_z": -80.84375,
+ "stations": "Swanson Vision,Thurston Holdings"
+ },
+ {
+ "name": "HIP 23824",
+ "pos_x": 121.5,
+ "pos_y": -78.125,
+ "pos_z": 60.84375,
+ "stations": "McKean Hub,Arnold Orbital,Fox Station,Naboth Dock,Nahavandi Station,Alexander Port,Isaev Port"
+ },
+ {
+ "name": "HIP 23857",
+ "pos_x": 165.3125,
+ "pos_y": -124.21875,
+ "pos_z": -26.0625,
+ "stations": "Crossfield Colony,Ashman Platform"
+ },
+ {
+ "name": "HIP 23869",
+ "pos_x": -84.65625,
+ "pos_y": 43.3125,
+ "pos_z": -109.6875,
+ "stations": "Wilcutt Settlement,Wohler Beacon"
+ },
+ {
+ "name": "HIP 23913",
+ "pos_x": -62.375,
+ "pos_y": 21.4375,
+ "pos_z": -146.09375,
+ "stations": "Hooke Prospect"
+ },
+ {
+ "name": "HIP 23923",
+ "pos_x": 130.40625,
+ "pos_y": -103.6875,
+ "pos_z": -58.78125,
+ "stations": "Hollander Colony,Westerhout Platform"
+ },
+ {
+ "name": "HIP 23926",
+ "pos_x": 128.8125,
+ "pos_y": -102.40625,
+ "pos_z": -58.0625,
+ "stations": "Lalande Survey"
+ },
+ {
+ "name": "HIP 23984",
+ "pos_x": 178.46875,
+ "pos_y": -125.9375,
+ "pos_z": 16.28125,
+ "stations": "Miyasaka Landing,Kandrup Orbital,Huss Mines"
+ },
+ {
+ "name": "HIP 24019",
+ "pos_x": -10.09375,
+ "pos_y": -20.09375,
+ "pos_z": -177.03125,
+ "stations": "von Bellingshausen Mines"
+ },
+ {
+ "name": "HIP 24020",
+ "pos_x": -10.09375,
+ "pos_y": -20.09375,
+ "pos_z": -177.03125,
+ "stations": "Evans Relay,Leclerc Base,Ito Base,Guidoni Hub"
+ },
+ {
+ "name": "HIP 24035",
+ "pos_x": -6.53125,
+ "pos_y": -15,
+ "pos_z": -128.375,
+ "stations": "Delbruck Camp"
+ },
+ {
+ "name": "HIP 24046",
+ "pos_x": -6.4375,
+ "pos_y": -15.21875,
+ "pos_z": -129.53125,
+ "stations": "Resnik Dock,Shull Port,Thirsk Hub,Baird Dock,Brand Hub,Hopkins Hub,Poindexter Terminal,Harris' Progress,Kelleam Prospect"
+ },
+ {
+ "name": "HIP 24084",
+ "pos_x": 209.4375,
+ "pos_y": -136.6875,
+ "pos_z": 88.125,
+ "stations": "Rosenberger's Inheritance,Leberecht Tempel Outpost"
+ },
+ {
+ "name": "HIP 24133",
+ "pos_x": -15.3125,
+ "pos_y": -9.4375,
+ "pos_z": -137.28125,
+ "stations": "Kelly Enterprise,Gaspar de Portola Arsenal,Gardner Beacon"
+ },
+ {
+ "name": "HIP 24157",
+ "pos_x": 127.15625,
+ "pos_y": -92.65625,
+ "pos_z": -13.125,
+ "stations": "Rabinowitz Dock,Coppel Horizons,Veron Relay"
+ },
+ {
+ "name": "HIP 24177",
+ "pos_x": 33.9375,
+ "pos_y": -47.34375,
+ "pos_z": -158.84375,
+ "stations": "Stokes' Claim,Sheckley Sanctuary,Merle Installation,Selberg Observatory"
+ },
+ {
+ "name": "HIP 2422",
+ "pos_x": -103.21875,
+ "pos_y": 31.34375,
+ "pos_z": -64.375,
+ "stations": "Spassky Station,Drake Orbital,Speke Port,Frobenius Hub,Yano Enterprise,Kovalevskaya Landing,Lindsey Depot"
+ },
+ {
+ "name": "HIP 24221",
+ "pos_x": 163.90625,
+ "pos_y": -114.4375,
+ "pos_z": 14.9375,
+ "stations": "Denning Vision,Napier Arsenal,Block Horizons"
+ },
+ {
+ "name": "HIP 24267",
+ "pos_x": 168.1875,
+ "pos_y": -121.46875,
+ "pos_z": -15,
+ "stations": "Luther Port,Tasman Enterprise,Fox Dock,Heinlein Penal colony"
+ },
+ {
+ "name": "HIP 24291",
+ "pos_x": 169.34375,
+ "pos_y": -132.625,
+ "pos_z": -90.375,
+ "stations": "Sorayama Port,Roberts Arsenal"
+ },
+ {
+ "name": "HIP 24316",
+ "pos_x": 180.5,
+ "pos_y": -128.40625,
+ "pos_z": -4.78125,
+ "stations": "Bok Survey,Peters Installation"
+ },
+ {
+ "name": "HIP 24320",
+ "pos_x": 152.90625,
+ "pos_y": -108.59375,
+ "pos_z": -2.9375,
+ "stations": "Flettner Landing"
+ },
+ {
+ "name": "HIP 24329",
+ "pos_x": -83.625,
+ "pos_y": 45.90625,
+ "pos_z": -96.28125,
+ "stations": "Cartmill Relay,Acharya Retreat,Nolan Settlement"
+ },
+ {
+ "name": "HIP 24336",
+ "pos_x": 39.78125,
+ "pos_y": -44.71875,
+ "pos_z": -121.90625,
+ "stations": "Baker Survey,Busch Works"
+ },
+ {
+ "name": "HIP 24344",
+ "pos_x": 137.21875,
+ "pos_y": -99.0625,
+ "pos_z": -15.6875,
+ "stations": "Artsutanov Station,Whelan Dock,Silva Bastion"
+ },
+ {
+ "name": "HIP 24424",
+ "pos_x": 121.84375,
+ "pos_y": -94.96875,
+ "pos_z": -70.6875,
+ "stations": "Sagan Freeport"
+ },
+ {
+ "name": "HIP 24463",
+ "pos_x": -40.5,
+ "pos_y": 10.3125,
+ "pos_z": -140.71875,
+ "stations": "al-Haytham Relay,Elcano Asylum"
+ },
+ {
+ "name": "HIP 2452",
+ "pos_x": 38.78125,
+ "pos_y": -209.28125,
+ "pos_z": 40.09375,
+ "stations": "Herschel Asylum"
+ },
+ {
+ "name": "HIP 2453",
+ "pos_x": -110.90625,
+ "pos_y": 16.5,
+ "pos_z": -67.84375,
+ "stations": "Lubin Ring,Yegorov Orbital,Koch Orbital,Maury Prospect"
+ },
+ {
+ "name": "HIP 24542",
+ "pos_x": 149,
+ "pos_y": -96.09375,
+ "pos_z": 64.6875,
+ "stations": "Wang Station,Stuart Works,Hague Port"
+ },
+ {
+ "name": "HIP 24597",
+ "pos_x": 115.65625,
+ "pos_y": -86.34375,
+ "pos_z": -47.8125,
+ "stations": "Chertovsky Settlement,Velho Escape"
+ },
+ {
+ "name": "HIP 24629",
+ "pos_x": 112.15625,
+ "pos_y": -87.5625,
+ "pos_z": -80.53125,
+ "stations": "Homer Enterprise,Blish Landing,Beliaev Silo,Schmidt Orbital"
+ },
+ {
+ "name": "HIP 24655",
+ "pos_x": -1.59375,
+ "pos_y": -14.65625,
+ "pos_z": -134.9375,
+ "stations": "Pinzon Beacon,Hedin Bastion,Hogan Dock"
+ },
+ {
+ "name": "HIP 24658",
+ "pos_x": -81.625,
+ "pos_y": 39.40625,
+ "pos_z": -147.625,
+ "stations": "Brady Hub"
+ },
+ {
+ "name": "HIP 24660",
+ "pos_x": 26.71875,
+ "pos_y": -37.96875,
+ "pos_z": -167.28125,
+ "stations": "Dall Enterprise,Bugrov Relay"
+ },
+ {
+ "name": "HIP 24681",
+ "pos_x": 38.5,
+ "pos_y": -42.875,
+ "pos_z": -140.21875,
+ "stations": "Nourse Platform,Herndon Base,Diamond Dock"
+ },
+ {
+ "name": "HIP 24720",
+ "pos_x": -79.40625,
+ "pos_y": 42.0625,
+ "pos_z": -113.1875,
+ "stations": "Pontes Landing,Savery Port,Carstensz Beacon"
+ },
+ {
+ "name": "HIP 24722",
+ "pos_x": 163.4375,
+ "pos_y": -106.75,
+ "pos_z": 55.53125,
+ "stations": "Ban Penal colony"
+ },
+ {
+ "name": "HIP 24777",
+ "pos_x": 66,
+ "pos_y": -55.65625,
+ "pos_z": -91.5625,
+ "stations": "Potagos Vision"
+ },
+ {
+ "name": "HIP 24864",
+ "pos_x": 40.3125,
+ "pos_y": -39.34375,
+ "pos_z": -110.8125,
+ "stations": "Vian Orbital,Guin Beacon"
+ },
+ {
+ "name": "HIP 24883",
+ "pos_x": 27.71875,
+ "pos_y": -34.9375,
+ "pos_z": -153.21875,
+ "stations": "Velho Terminal,Herjulfsson Dock,Bushkov Terminal,Kovalevskaya Relay"
+ },
+ {
+ "name": "HIP 24912",
+ "pos_x": 149.6875,
+ "pos_y": -112.8125,
+ "pos_z": -100.6875,
+ "stations": "Palmer's Pride,Poincare Mines"
+ },
+ {
+ "name": "HIP 24923",
+ "pos_x": 37.90625,
+ "pos_y": -44.21875,
+ "pos_z": -178.65625,
+ "stations": "Hennepin Platform"
+ },
+ {
+ "name": "HIP 24947",
+ "pos_x": 118.5625,
+ "pos_y": -86.84375,
+ "pos_z": -56.84375,
+ "stations": "Eisenstein Hub,Rabinowitz Plant,Heiles Landing,Garrett Settlement,Hansen Prospect"
+ },
+ {
+ "name": "HIP 24968",
+ "pos_x": 219.71875,
+ "pos_y": -151.78125,
+ "pos_z": -16.84375,
+ "stations": "Pimi Terminal,Bainbridge Station,Hyecho Holdings,Bennett Gateway,Riess Depot"
+ },
+ {
+ "name": "HIP 24986",
+ "pos_x": 217.40625,
+ "pos_y": -146.875,
+ "pos_z": 15.3125,
+ "stations": "Flagg Relay"
+ },
+ {
+ "name": "HIP 2504",
+ "pos_x": 79.15625,
+ "pos_y": -205.84375,
+ "pos_z": 63.65625,
+ "stations": "Mather Beacon"
+ },
+ {
+ "name": "HIP 2511",
+ "pos_x": -6.40625,
+ "pos_y": -221.09375,
+ "pos_z": 13.3125,
+ "stations": "De Colony,Jean Prospect,Alvarado Installation"
+ },
+ {
+ "name": "HIP 25190",
+ "pos_x": 129.25,
+ "pos_y": -93.65625,
+ "pos_z": -71.9375,
+ "stations": "van den Bergh Station,Baily Vision,Corben Port,Sarmiento de Gamboa Base"
+ },
+ {
+ "name": "HIP 25191",
+ "pos_x": 73.0625,
+ "pos_y": -63.25,
+ "pos_z": -157.53125,
+ "stations": "Payson Platform,Schroeder's Claim"
+ },
+ {
+ "name": "HIP 25195",
+ "pos_x": 21.28125,
+ "pos_y": -28.09375,
+ "pos_z": -156.375,
+ "stations": "Person Keep,Soper Vista"
+ },
+ {
+ "name": "HIP 25224",
+ "pos_x": -61.8125,
+ "pos_y": 27.28125,
+ "pos_z": -166.21875,
+ "stations": "Narbeth Colony,Csoma Point"
+ },
+ {
+ "name": "HIP 25231",
+ "pos_x": 185.65625,
+ "pos_y": -124.625,
+ "pos_z": 6.125,
+ "stations": "Janifer Installation,Brouwer Works"
+ },
+ {
+ "name": "HIP 2533",
+ "pos_x": -53.5625,
+ "pos_y": -138.875,
+ "pos_z": -21.65625,
+ "stations": "Bauman Station"
+ },
+ {
+ "name": "HIP 25359",
+ "pos_x": 173.96875,
+ "pos_y": -121.34375,
+ "pos_z": -63.03125,
+ "stations": "Dassault Vision,Bolton Vision,Anderson Installation"
+ },
+ {
+ "name": "HIP 25393",
+ "pos_x": -70.125,
+ "pos_y": 37.46875,
+ "pos_z": -122.53125,
+ "stations": "Crown Colony,Duffy's Folly"
+ },
+ {
+ "name": "HIP 25396",
+ "pos_x": 86.15625,
+ "pos_y": -64.65625,
+ "pos_z": -94.4375,
+ "stations": "Peirce Enterprise,Dugan Station"
+ },
+ {
+ "name": "HIP 2544",
+ "pos_x": -18.5,
+ "pos_y": -245,
+ "pos_z": 7.28125,
+ "stations": "Carnera Port,Sy Vision"
+ },
+ {
+ "name": "HIP 25461",
+ "pos_x": 134.5625,
+ "pos_y": -92.875,
+ "pos_z": -46.75,
+ "stations": "August von Steinheil Ring"
+ },
+ {
+ "name": "HIP 25490",
+ "pos_x": 130,
+ "pos_y": -92.625,
+ "pos_z": -90,
+ "stations": "Cousin's Claim"
+ },
+ {
+ "name": "HIP 25506",
+ "pos_x": 162.9375,
+ "pos_y": -111.78125,
+ "pos_z": -52.625,
+ "stations": "Cesar Janssen Dock,Timofeyevich Laboratory,Carver Beacon,Adams Vision"
+ },
+ {
+ "name": "HIP 25559",
+ "pos_x": 82.90625,
+ "pos_y": -59.1875,
+ "pos_z": -64.125,
+ "stations": "Cantor Market,Russell Terminal,Hughes Enterprise,Eskridge Station,Yu City,Hyecho Terminal,Stillman Enterprise,Vonarburg Works,Fancher Depot"
+ },
+ {
+ "name": "HIP 2563",
+ "pos_x": -134.78125,
+ "pos_y": -104.59375,
+ "pos_z": -73.53125,
+ "stations": "Fernandes de Queiros Landing"
+ },
+ {
+ "name": "HIP 25677",
+ "pos_x": 42.5625,
+ "pos_y": -38.28125,
+ "pos_z": -166.96875,
+ "stations": "Linnehan Station,Arkwright Port"
+ },
+ {
+ "name": "HIP 25679",
+ "pos_x": 97.3125,
+ "pos_y": -70.625,
+ "pos_z": -107.65625,
+ "stations": "Zahn Plant,Legendre Orbital,Hambly Hub"
+ },
+ {
+ "name": "HIP 2574",
+ "pos_x": 22.84375,
+ "pos_y": -261.6875,
+ "pos_z": 33.125,
+ "stations": "Lagerkvist Colony"
+ },
+ {
+ "name": "HIP 25746",
+ "pos_x": 136.5,
+ "pos_y": -91.84375,
+ "pos_z": -40.90625,
+ "stations": "Alden Colony,Kushida Terminal"
+ },
+ {
+ "name": "HIP 25759",
+ "pos_x": 210.625,
+ "pos_y": -137.59375,
+ "pos_z": 6.125,
+ "stations": "Jones Vision,Kirby Station,Jefferies Station"
+ },
+ {
+ "name": "HIP 25795",
+ "pos_x": 106.46875,
+ "pos_y": -72.71875,
+ "pos_z": -56.9375,
+ "stations": "Fuchs Freeport,Wallis Installation,Nicholson's Exile"
+ },
+ {
+ "name": "HIP 25843",
+ "pos_x": 110.71875,
+ "pos_y": -76.65625,
+ "pos_z": -85.78125,
+ "stations": "Henry Port,Barnard Survey,Popper Terminal"
+ },
+ {
+ "name": "HIP 25860",
+ "pos_x": 27.3125,
+ "pos_y": -25.90625,
+ "pos_z": -158.09375,
+ "stations": "Chaudhary Landing"
+ },
+ {
+ "name": "HIP 25879",
+ "pos_x": 221.90625,
+ "pos_y": -143.6875,
+ "pos_z": 14,
+ "stations": "Wylie Prospect,Delporte Settlement,Apianus Port"
+ },
+ {
+ "name": "HIP 25880",
+ "pos_x": -49.28125,
+ "pos_y": 26.25,
+ "pos_z": -115.4375,
+ "stations": "Ramsay City"
+ },
+ {
+ "name": "HIP 25963",
+ "pos_x": 155.90625,
+ "pos_y": -103.125,
+ "pos_z": -45.15625,
+ "stations": "Fehrenbach Station,Roelofs Hub,Edwards Dock"
+ },
+ {
+ "name": "HIP 26018",
+ "pos_x": 16.53125,
+ "pos_y": -16.9375,
+ "pos_z": -147.34375,
+ "stations": "Benz Colony,Foda Terminal"
+ },
+ {
+ "name": "HIP 26030",
+ "pos_x": 172.53125,
+ "pos_y": -110.75,
+ "pos_z": 14.15625,
+ "stations": "Leuschner Landing,Okuni Vision,Henry Port,Pinto Refinery"
+ },
+ {
+ "name": "HIP 26037",
+ "pos_x": -31.71875,
+ "pos_y": 12.9375,
+ "pos_z": -180.34375,
+ "stations": "Tucker Landing,Grimwood Landing,Arnarson Landing"
+ },
+ {
+ "name": "HIP 26067",
+ "pos_x": 113.875,
+ "pos_y": -74.34375,
+ "pos_z": -23.96875,
+ "stations": "Leberecht Tempel Beacon,Jung Barracks"
+ },
+ {
+ "name": "HIP 26144",
+ "pos_x": 214.5,
+ "pos_y": -137.8125,
+ "pos_z": -4.3125,
+ "stations": "Yerka Mine,Mukai Point,Muhammad Ibn Battuta Base"
+ },
+ {
+ "name": "HIP 26269",
+ "pos_x": 126.4375,
+ "pos_y": -78.96875,
+ "pos_z": 55.8125,
+ "stations": "Yamamoto Port"
+ },
+ {
+ "name": "HIP 26274",
+ "pos_x": 81.34375,
+ "pos_y": -56.03125,
+ "pos_z": -134.34375,
+ "stations": "Schachner's Progress,Tavares' Folly"
+ },
+ {
+ "name": "HIP 26372",
+ "pos_x": -126.5,
+ "pos_y": 76.5625,
+ "pos_z": -145.78125,
+ "stations": "Nixon Oasis"
+ },
+ {
+ "name": "HIP 26392",
+ "pos_x": -28.71875,
+ "pos_y": 14.8125,
+ "pos_z": -140.46875,
+ "stations": "Werner von Siemens Station,Kirchoff City,Karl Diesel Station,Kanwar Orbital,England Enterprise"
+ },
+ {
+ "name": "HIP 2645",
+ "pos_x": 29.84375,
+ "pos_y": -229.375,
+ "pos_z": 34.15625,
+ "stations": "Fowler Landing,Suzuki Colony,Juan de la Cierva Colony"
+ },
+ {
+ "name": "HIP 26651",
+ "pos_x": 11.21875,
+ "pos_y": -9.09375,
+ "pos_z": -174.6875,
+ "stations": "Offutt Dock"
+ },
+ {
+ "name": "HIP 26688",
+ "pos_x": 101.6875,
+ "pos_y": -64.5625,
+ "pos_z": -107.90625,
+ "stations": "Hadamard's Inheritance"
+ },
+ {
+ "name": "HIP 26689",
+ "pos_x": 31.75,
+ "pos_y": -21.53125,
+ "pos_z": -172.59375,
+ "stations": "Converse's Progress,Heceta's Inheritance"
+ },
+ {
+ "name": "HIP 26761",
+ "pos_x": 12,
+ "pos_y": -8.625,
+ "pos_z": -184.625,
+ "stations": "Shosuke Orbital,McHugh Colony,Morgan Keep"
+ },
+ {
+ "name": "HIP 26830",
+ "pos_x": 149,
+ "pos_y": -92.5,
+ "pos_z": -34.34375,
+ "stations": "Gloss Vision,Banneker Terminal,Asprin Depot,Webb Vision"
+ },
+ {
+ "name": "HIP 26883",
+ "pos_x": 136.53125,
+ "pos_y": -84.40625,
+ "pos_z": -30.09375,
+ "stations": "Verrier Stop,Emshwiller's Folly,Somayaji Vision,Gaughan Hub"
+ },
+ {
+ "name": "HIP 26890",
+ "pos_x": -73.625,
+ "pos_y": 45.5,
+ "pos_z": -136.40625,
+ "stations": "Rubruck Dock,Roberts Arena"
+ },
+ {
+ "name": "HIP 26926",
+ "pos_x": 81.0625,
+ "pos_y": -49.75,
+ "pos_z": -132.3125,
+ "stations": "Stevens Orbital,Wagner City,Perga Hub,Wiener Dock"
+ },
+ {
+ "name": "HIP 26933",
+ "pos_x": -50.71875,
+ "pos_y": 31.5625,
+ "pos_z": -126.15625,
+ "stations": "Beckman Hangar,Acaba Hangar,Lenthall Barracks,Carey Orbital"
+ },
+ {
+ "name": "HIP 26985",
+ "pos_x": 120,
+ "pos_y": -74,
+ "pos_z": 39.59375,
+ "stations": "Adams Port,Reinmuth Terminal,Zwicky Dock"
+ },
+ {
+ "name": "HIP 26990",
+ "pos_x": 143.625,
+ "pos_y": -87.96875,
+ "pos_z": -65.21875,
+ "stations": "Anthony Orbital,Cook Dock,Yourkevitch Orbital,Bailey Beacon,Kelly Barracks"
+ },
+ {
+ "name": "HIP 27058",
+ "pos_x": 71.9375,
+ "pos_y": -43.25,
+ "pos_z": -103.90625,
+ "stations": "Carrasco Ring,Jakes Dock,Panshin Base,Salgari Station,Plante Dock,Jones Orbital,Levinson Terminal,Hieb Town,Makarov Port,Ahmed Gateway"
+ },
+ {
+ "name": "HIP 27067",
+ "pos_x": -28.40625,
+ "pos_y": 18.84375,
+ "pos_z": -167.71875,
+ "stations": "Tennyson d'Eyncourt Colony,Drummond Base,Bisson Terminal"
+ },
+ {
+ "name": "HIP 2710",
+ "pos_x": -44.40625,
+ "pos_y": -124.46875,
+ "pos_z": -18.8125,
+ "stations": "Sevastyanov Port"
+ },
+ {
+ "name": "HIP 27123",
+ "pos_x": -11.5,
+ "pos_y": 9.09375,
+ "pos_z": -176.78125,
+ "stations": "Silva Camp,Thuot Relay,Minkowski Point"
+ },
+ {
+ "name": "HIP 27134",
+ "pos_x": 138.71875,
+ "pos_y": -84.59375,
+ "pos_z": -3.3125,
+ "stations": "Reichelt Station,Brom Port,Joseph Delambre Vision,Nilson Dock,Asclepi Port,Babcock's Progress,Lanier Refinery,Burnham Landing,Foden Landing"
+ },
+ {
+ "name": "HIP 27155",
+ "pos_x": -16.28125,
+ "pos_y": 12.15625,
+ "pos_z": -172.125,
+ "stations": "Fadlan Hub,Makarov Hub"
+ },
+ {
+ "name": "HIP 27185",
+ "pos_x": 123.375,
+ "pos_y": -73,
+ "pos_z": -144.40625,
+ "stations": "Steiner Enterprise,Harper's Claim,Artin Hub"
+ },
+ {
+ "name": "HIP 2725",
+ "pos_x": -84.96875,
+ "pos_y": -71.71875,
+ "pos_z": -47.15625,
+ "stations": "Legendre Orbital,Bentham Terminal,Kiernan City,Kiernan Dock,Lefschetz Gateway,Marques Keep,Noli Terminal,Roggeveen Holdings"
+ },
+ {
+ "name": "HIP 27305",
+ "pos_x": 112.53125,
+ "pos_y": -64.78125,
+ "pos_z": -157.15625,
+ "stations": "Russell Port,Ashton Installation,Makarov Dock"
+ },
+ {
+ "name": "HIP 27330",
+ "pos_x": 127.53125,
+ "pos_y": -74.84375,
+ "pos_z": -99.53125,
+ "stations": "Al-Kashi Depot"
+ },
+ {
+ "name": "HIP 2736",
+ "pos_x": -70.90625,
+ "pos_y": -126.84375,
+ "pos_z": -35.15625,
+ "stations": "H. G. Wells Settlement,Jolliet Vision"
+ },
+ {
+ "name": "HIP 27371",
+ "pos_x": 117.03125,
+ "pos_y": -69.28125,
+ "pos_z": -52.25,
+ "stations": "Williams Station,Crown Terminal,Brillant Station,Cousin Keep,Laumer Beacon"
+ },
+ {
+ "name": "HIP 27417",
+ "pos_x": 98.09375,
+ "pos_y": -56.78125,
+ "pos_z": -84.1875,
+ "stations": "Blair Port,Penn Enterprise,al-Khayyam Terminal,Guerrero Gateway,Hamilton Enterprise,Kondo Barracks,Bering Dock,Anthony Enterprise,Weber Vision,Albategnius Enterprise"
+ },
+ {
+ "name": "HIP 2747",
+ "pos_x": 50.875,
+ "pos_y": -164.96875,
+ "pos_z": 41.5,
+ "stations": "Chacornac Platform,Goodman Hub,Vance Depot"
+ },
+ {
+ "name": "HIP 27578",
+ "pos_x": 138.59375,
+ "pos_y": -80.6875,
+ "pos_z": -50.15625,
+ "stations": "Sukhoi Hub,Lockyer Orbital,Reis Landing,Mustelin Port,Sekowski Mine"
+ },
+ {
+ "name": "HIP 27609",
+ "pos_x": 119.9375,
+ "pos_y": -68.09375,
+ "pos_z": -86,
+ "stations": "Bottego Platform"
+ },
+ {
+ "name": "HIP 2761",
+ "pos_x": 131.375,
+ "pos_y": -255.65625,
+ "pos_z": 96.21875,
+ "stations": "Marsden Camp,Naubakht Works"
+ },
+ {
+ "name": "HIP 27633",
+ "pos_x": 162.90625,
+ "pos_y": -97.8125,
+ "pos_z": 29.5,
+ "stations": "Impey Base"
+ },
+ {
+ "name": "HIP 277",
+ "pos_x": 10.34375,
+ "pos_y": -208.46875,
+ "pos_z": 47.34375,
+ "stations": "Carpini Base,Tomita Colony,Sagan Platform"
+ },
+ {
+ "name": "HIP 27758",
+ "pos_x": 82.125,
+ "pos_y": -41.65625,
+ "pos_z": -151.5,
+ "stations": "Rontgen Mine,Shull Dock,Stafford Prospect"
+ },
+ {
+ "name": "HIP 27823",
+ "pos_x": 131.9375,
+ "pos_y": -74.125,
+ "pos_z": -69.84375,
+ "stations": "Baillaud Vision,Anastase Perrotin Terminal,Parsons Dock,McKie Barracks"
+ },
+ {
+ "name": "HIP 27855",
+ "pos_x": 189.28125,
+ "pos_y": -110.125,
+ "pos_z": -16.78125,
+ "stations": "Burkin Settlement,Mouchez Dock,Dumont Dock"
+ },
+ {
+ "name": "HIP 27894",
+ "pos_x": 118.5625,
+ "pos_y": -65.65625,
+ "pos_z": -70.6875,
+ "stations": "McNair Colony,Lu Settlement"
+ },
+ {
+ "name": "HIP 27923",
+ "pos_x": 206.1875,
+ "pos_y": -119.15625,
+ "pos_z": -21.6875,
+ "stations": "van den Bergh Colony"
+ },
+ {
+ "name": "HIP 27948",
+ "pos_x": 157.34375,
+ "pos_y": -92.375,
+ "pos_z": 12.96875,
+ "stations": "Richer Landing,Oren Platform,Dubyago Terminal,Gregory Vision"
+ },
+ {
+ "name": "HIP 27957",
+ "pos_x": 161.5,
+ "pos_y": -96.75,
+ "pos_z": 48.3125,
+ "stations": "Anderson Platform"
+ },
+ {
+ "name": "HIP 27969",
+ "pos_x": 198,
+ "pos_y": -116.375,
+ "pos_z": 19.71875,
+ "stations": "Angstrom Landing"
+ },
+ {
+ "name": "HIP 27980",
+ "pos_x": 59.96875,
+ "pos_y": -28.40625,
+ "pos_z": -116.9375,
+ "stations": "Ramon Terminal,Planck Ring,Hammond Gateway,Sharman Terminal,Ross Enterprise,Barba Ring,Ham Hub,Liska Dock,Bobko Dock,Stewart Hub,Weierstrass Enterprise"
+ },
+ {
+ "name": "HIP 27986",
+ "pos_x": 117.34375,
+ "pos_y": -59.125,
+ "pos_z": -163.15625,
+ "stations": "Stevens Dock,Webb Port,Bond Port,Kurland Vista"
+ },
+ {
+ "name": "HIP 28036",
+ "pos_x": 143.03125,
+ "pos_y": -78.9375,
+ "pos_z": -69.0625,
+ "stations": "Giacobini Oasis,Simak Plant,May Colony,Fisk Arsenal"
+ },
+ {
+ "name": "HIP 28070",
+ "pos_x": 188.15625,
+ "pos_y": -112.5,
+ "pos_z": 58.84375,
+ "stations": "Courvoisier Port,Yu Installation,Carver's Pride"
+ },
+ {
+ "name": "HIP 28122",
+ "pos_x": 41.28125,
+ "pos_y": -15.6875,
+ "pos_z": -130.78125,
+ "stations": "Kaku Ring,Henry Terminal,Melroy Station,Goldstein Barracks,Clark Installation"
+ },
+ {
+ "name": "HIP 28135",
+ "pos_x": 40.1875,
+ "pos_y": -15.1875,
+ "pos_z": -126.65625,
+ "stations": "Ford Orbital"
+ },
+ {
+ "name": "HIP 28150",
+ "pos_x": 147.9375,
+ "pos_y": -82.03125,
+ "pos_z": -51.71875,
+ "stations": "Richer Platform,Allen Prospect,Ikeya Prospect,Borrelly Beacon,Taylor Installation"
+ },
+ {
+ "name": "HIP 28193",
+ "pos_x": 109.3125,
+ "pos_y": -59.34375,
+ "pos_z": -53.1875,
+ "stations": "Michalitsianos Hub,Dolgov Penal colony"
+ },
+ {
+ "name": "HIP 2824",
+ "pos_x": -112.375,
+ "pos_y": -84.78125,
+ "pos_z": -63.78125,
+ "stations": "Horowitz Dock,Euthymenes Silo,Lukyanenko Relay"
+ },
+ {
+ "name": "HIP 2828",
+ "pos_x": -88.0625,
+ "pos_y": -118.375,
+ "pos_z": -46.90625,
+ "stations": "Hurston Gateway,Lopez de Villalobos Prospect,Kirtley Bastion"
+ },
+ {
+ "name": "HIP 2841",
+ "pos_x": 80.15625,
+ "pos_y": -156.3125,
+ "pos_z": 58.34375,
+ "stations": "Hagihara Landing,Kalam Camp,Tsiolkovsky Installation"
+ },
+ {
+ "name": "HIP 2843",
+ "pos_x": -50.25,
+ "pos_y": -136.1875,
+ "pos_z": -22.78125,
+ "stations": "Rennie Dock,Hubble Survey"
+ },
+ {
+ "name": "HIP 28452",
+ "pos_x": 135.09375,
+ "pos_y": -76.46875,
+ "pos_z": -1.40625,
+ "stations": "Chernykh Hub,Iben Hub,Barr Silo"
+ },
+ {
+ "name": "HIP 28460",
+ "pos_x": 148.625,
+ "pos_y": -83.0625,
+ "pos_z": -14.40625,
+ "stations": "Grant Survey,Pickering Port,Land Barracks,Condit Colony"
+ },
+ {
+ "name": "HIP 2847",
+ "pos_x": -86.3125,
+ "pos_y": -140.34375,
+ "pos_z": -44.65625,
+ "stations": "Reynolds Port,Hopkins Dock,H. G. Wells Plant"
+ },
+ {
+ "name": "HIP 28498",
+ "pos_x": 158.5625,
+ "pos_y": -88.09375,
+ "pos_z": -19.1875,
+ "stations": "Froud Terminal,Farghani Vision,Staden Refinery"
+ },
+ {
+ "name": "HIP 28525",
+ "pos_x": 217.59375,
+ "pos_y": -129.96875,
+ "pos_z": 86.375,
+ "stations": "Maupertuis Relay"
+ },
+ {
+ "name": "HIP 28573",
+ "pos_x": 184.15625,
+ "pos_y": -107.15625,
+ "pos_z": 41.15625,
+ "stations": "van Houten Depot,Dietz Beacon,Arago Terminal"
+ },
+ {
+ "name": "HIP 28604",
+ "pos_x": 141.28125,
+ "pos_y": -75.125,
+ "pos_z": -48.71875,
+ "stations": "Borrelly Station,Palitzsch Survey,Kepler Landing"
+ },
+ {
+ "name": "HIP 28626",
+ "pos_x": -2.6875,
+ "pos_y": 15.46875,
+ "pos_z": -157.875,
+ "stations": "Moore Mines,Archer Survey,Grijalva Arsenal"
+ },
+ {
+ "name": "HIP 28644",
+ "pos_x": -2.65625,
+ "pos_y": 15.3125,
+ "pos_z": -154.3125,
+ "stations": "Hennepin Bastion,Lefschetz's Folly,la Cosa Horizons"
+ },
+ {
+ "name": "HIP 28678",
+ "pos_x": 133.625,
+ "pos_y": -61.34375,
+ "pos_z": -145.84375,
+ "stations": "Webb Settlement,Bethke Landing,Giles Stop,Plucker Arsenal"
+ },
+ {
+ "name": "HIP 28774",
+ "pos_x": 1.84375,
+ "pos_y": 6.96875,
+ "pos_z": -82.9375,
+ "stations": "Lucid Port,Belyanin Relay,Lonchakov Bastion,Linteris Port,Wittgenstein Enterprise,Oefelein Dock"
+ },
+ {
+ "name": "HIP 2881",
+ "pos_x": 120.15625,
+ "pos_y": -221.84375,
+ "pos_z": 86.40625,
+ "stations": "McMullen Plant,Anderson Port,Dyson Station"
+ },
+ {
+ "name": "HIP 28813",
+ "pos_x": 35.03125,
+ "pos_y": -6.6875,
+ "pos_z": -129.25,
+ "stations": "Gibson Camp,Dozois Enterprise,Schouten Ring,Gabriel Landing,Carr Orbital,Kerr Station,Rodrigues Arsenal"
+ },
+ {
+ "name": "HIP 28869",
+ "pos_x": 130.34375,
+ "pos_y": -72.1875,
+ "pos_z": 2.03125,
+ "stations": "Grant Prospect,Tank Silo,Tietjen Arena,Chelomey Dock"
+ },
+ {
+ "name": "HIP 28987",
+ "pos_x": 130.71875,
+ "pos_y": -67.59375,
+ "pos_z": -38.5625,
+ "stations": "Wallis Orbital,Chernykh Orbital,Piano Port"
+ },
+ {
+ "name": "HIP 290",
+ "pos_x": -6.625,
+ "pos_y": -207.8125,
+ "pos_z": 38.15625,
+ "stations": "Ahnert Colony,Bell Port,Dolgov Port,Scithers Point"
+ },
+ {
+ "name": "HIP 2902",
+ "pos_x": -15.90625,
+ "pos_y": -231.9375,
+ "pos_z": 3.125,
+ "stations": "Cassini City,Schwarzschild Port,Clapperton Keep"
+ },
+ {
+ "name": "HIP 2909",
+ "pos_x": -13.125,
+ "pos_y": -191.40625,
+ "pos_z": 2.5,
+ "stations": "Reinhold Terminal,Henry Colony"
+ },
+ {
+ "name": "HIP 29096",
+ "pos_x": 107.1875,
+ "pos_y": -50.1875,
+ "pos_z": -73.28125,
+ "stations": "Chiao Ring,Anthony Vista"
+ },
+ {
+ "name": "HIP 29241",
+ "pos_x": 119.125,
+ "pos_y": -53.40625,
+ "pos_z": -91.625,
+ "stations": "Rozhdestvensky Enterprise,Zhen Orbital,Gresley Gateway,Stasheff Keep"
+ },
+ {
+ "name": "HIP 293",
+ "pos_x": 98,
+ "pos_y": -232.25,
+ "pos_z": 98,
+ "stations": "Yanai Orbital,Nahavandi Vision"
+ },
+ {
+ "name": "HIP 29312",
+ "pos_x": 18.3125,
+ "pos_y": 7.65625,
+ "pos_z": -141.75,
+ "stations": "Ohm Horizons,Morgan Orbital"
+ },
+ {
+ "name": "HIP 29314",
+ "pos_x": 220.46875,
+ "pos_y": -118.90625,
+ "pos_z": 2.625,
+ "stations": "Furuta Dock,Tshang Vision,Prandtl Lab"
+ },
+ {
+ "name": "HIP 29439",
+ "pos_x": 200.21875,
+ "pos_y": -109.34375,
+ "pos_z": 18.4375,
+ "stations": "van de Hulst Outpost,Godwin Point"
+ },
+ {
+ "name": "HIP 29444",
+ "pos_x": 147.375,
+ "pos_y": -80.46875,
+ "pos_z": 13.5625,
+ "stations": "Swift Vision,Gehry Dock,Jones Station,Lehtonen Relay,Herreshoff Silo"
+ },
+ {
+ "name": "HIP 29582",
+ "pos_x": -13.71875,
+ "pos_y": 26.875,
+ "pos_z": -143.40625,
+ "stations": "Babcock Prospect,Arnold Horizons"
+ },
+ {
+ "name": "HIP 29596",
+ "pos_x": 165.25,
+ "pos_y": -82.4375,
+ "pos_z": -35.75,
+ "stations": "Brule Arsenal,de Kamp Vision,Walotsky Hub,Adelman Dock,Roberts Beacon"
+ },
+ {
+ "name": "HIP 296",
+ "pos_x": -9.28125,
+ "pos_y": -124.96875,
+ "pos_z": 20.125,
+ "stations": "Nakamura City,Richer City,Wang Ring,Opik Vision,Malvern Gateway"
+ },
+ {
+ "name": "HIP 29644",
+ "pos_x": 111.59375,
+ "pos_y": -44.3125,
+ "pos_z": -103.4375,
+ "stations": "Williamson Settlement,Puleston Beacon"
+ },
+ {
+ "name": "HIP 29716",
+ "pos_x": 59.34375,
+ "pos_y": -16.15625,
+ "pos_z": -104.5625,
+ "stations": "Halley Ring,Burgess Installation,Scully-Power Enterprise,Patrick Installation,Brule Beacon"
+ },
+ {
+ "name": "HIP 29720",
+ "pos_x": -61.03125,
+ "pos_y": 46.3125,
+ "pos_z": -99.5625,
+ "stations": "Dias Hub,Watts Dock,Reamy Gateway,Merchiston Enterprise,Cleve Station,Peral Dock,Baxter Orbital"
+ },
+ {
+ "name": "HIP 29777",
+ "pos_x": -57.53125,
+ "pos_y": 50.59375,
+ "pos_z": -140.25,
+ "stations": "Forfait Point,Nourse Beacon,Chalker Depot"
+ },
+ {
+ "name": "HIP 29792",
+ "pos_x": 146,
+ "pos_y": -85.6875,
+ "pos_z": 63.75,
+ "stations": "Vaucouleurs Dock,Carsono Ring,Swift Gateway,Daniel Plant"
+ },
+ {
+ "name": "HIP 29804",
+ "pos_x": 105.1875,
+ "pos_y": -48.6875,
+ "pos_z": -42.4375,
+ "stations": "Heaviside Platform"
+ },
+ {
+ "name": "HIP 29829",
+ "pos_x": 124.3125,
+ "pos_y": -47.125,
+ "pos_z": -118.71875,
+ "stations": "Fernandez Mines,O'Donnell Mine"
+ },
+ {
+ "name": "HIP 29964",
+ "pos_x": 107.96875,
+ "pos_y": -59.625,
+ "pos_z": 24.3125,
+ "stations": "Burnham Holdings,Sandage Platform"
+ },
+ {
+ "name": "HIP 30001",
+ "pos_x": 144.4375,
+ "pos_y": -59,
+ "pos_z": -98.65625,
+ "stations": "Dunning's Town"
+ },
+ {
+ "name": "HIP 30030",
+ "pos_x": 85.6875,
+ "pos_y": -22.9375,
+ "pos_z": -133.875,
+ "stations": "Aksyonov Settlement,Donaldson Depot"
+ },
+ {
+ "name": "HIP 30038",
+ "pos_x": 169.53125,
+ "pos_y": -92.125,
+ "pos_z": 30.28125,
+ "stations": "Zacuto Holdings,Korolyov Works,Bouwens Relay"
+ },
+ {
+ "name": "HIP 30045",
+ "pos_x": 109.0625,
+ "pos_y": -48.0625,
+ "pos_z": -50.75,
+ "stations": "Kuchner Port,Reber Gateway"
+ },
+ {
+ "name": "HIP 30130",
+ "pos_x": -74.46875,
+ "pos_y": 58.625,
+ "pos_z": -125.53125,
+ "stations": "Bennett Terminal,Henry's Claim,Fearn Base"
+ },
+ {
+ "name": "HIP 30158",
+ "pos_x": 159.96875,
+ "pos_y": -73.46875,
+ "pos_z": -49.75,
+ "stations": "Cassini Prospect,O'Donnell's Progress,Shapley Terminal,Yanai Platform,Oikawa Installation"
+ },
+ {
+ "name": "HIP 30179",
+ "pos_x": 165.5625,
+ "pos_y": -94.46875,
+ "pos_z": 60,
+ "stations": "Harvey-Smith Oasis,Borrelly Terminal,Obruchev Prospect"
+ },
+ {
+ "name": "HIP 30188",
+ "pos_x": 11.59375,
+ "pos_y": 23.5,
+ "pos_z": -176.59375,
+ "stations": "Alenquer Enterprise"
+ },
+ {
+ "name": "HIP 3020",
+ "pos_x": 65.6875,
+ "pos_y": -250.09375,
+ "pos_z": 52.71875,
+ "stations": "Dunlop Landing,McAllaster Arsenal"
+ },
+ {
+ "name": "HIP 30220",
+ "pos_x": 32.8125,
+ "pos_y": 4.59375,
+ "pos_z": -126.75,
+ "stations": "Wankel Escape,Midgeley Terminal"
+ },
+ {
+ "name": "HIP 30243",
+ "pos_x": 65.65625,
+ "pos_y": -14.375,
+ "pos_z": -112.25,
+ "stations": "Piper Station,Platt Terminal,Drexler Dock,Smith Port,Issigonis Enterprise,Hewish Orbital,Poleshchuk Port,Song Station,Boas Orbital,Sekelj Penal colony,Ings Reach,Coulter Terminal,Walker Base,Ansari Holdings"
+ },
+ {
+ "name": "HIP 30252",
+ "pos_x": 207.625,
+ "pos_y": -99,
+ "pos_z": -38,
+ "stations": "Poyser Colony,Adams Arsenal"
+ },
+ {
+ "name": "HIP 30260",
+ "pos_x": 156.15625,
+ "pos_y": -68.90625,
+ "pos_z": -60.8125,
+ "stations": "Hay Port,Charlier City,Clark Arsenal"
+ },
+ {
+ "name": "HIP 3028",
+ "pos_x": -33.46875,
+ "pos_y": -132.0625,
+ "pos_z": -14.46875,
+ "stations": "Laing Horizons,Wollheim Landing,Ross Colony,Read Terminal,Bretnor Landing"
+ },
+ {
+ "name": "HIP 3035",
+ "pos_x": -15.9375,
+ "pos_y": -204,
+ "pos_z": -0.21875,
+ "stations": "Wilhelm von Struve Station,Meuron Port,Hafner Vision,Mayor Base"
+ },
+ {
+ "name": "HIP 30486",
+ "pos_x": -10.53125,
+ "pos_y": 30.65625,
+ "pos_z": -140.3125,
+ "stations": "Cavendish Base,Ramelli Observatory"
+ },
+ {
+ "name": "HIP 305",
+ "pos_x": -11.125,
+ "pos_y": -150.65625,
+ "pos_z": 24.15625,
+ "stations": "Christian Base,Mohr Port,Leopold Heckmann Vision,Bessel Port"
+ },
+ {
+ "name": "HIP 30502",
+ "pos_x": 170.0625,
+ "pos_y": -74.6875,
+ "pos_z": -56.90625,
+ "stations": "Tennyson d'Eyncourt Vision,Harding Oasis,Clauss Base"
+ },
+ {
+ "name": "HIP 30509",
+ "pos_x": 105.96875,
+ "pos_y": -25.5625,
+ "pos_z": -150.65625,
+ "stations": "Lowry Terminal,Gibbs Holdings,al-Khayyam Silo"
+ },
+ {
+ "name": "HIP 306",
+ "pos_x": 90.6875,
+ "pos_y": -207.96875,
+ "pos_z": 89.125,
+ "stations": "Saha Hub,Juan de la Cierva Gateway,Merrill Terminal,Rothman Survey,Godwin Survey"
+ },
+ {
+ "name": "HIP 3062",
+ "pos_x": -139.75,
+ "pos_y": -91.875,
+ "pos_z": -82.53125,
+ "stations": "Huxley Outpost,Garrett Escape"
+ },
+ {
+ "name": "HIP 307",
+ "pos_x": 36.0625,
+ "pos_y": -234.6875,
+ "pos_z": 65.6875,
+ "stations": "Roche Hub,Deutsch Station,Lundmark Vision,Ziljak Lab,Petlyakov Keep,Dilworth Dock"
+ },
+ {
+ "name": "HIP 30733",
+ "pos_x": 105.0625,
+ "pos_y": -37.125,
+ "pos_z": -76.03125,
+ "stations": "Knight Ring,Tryggvason Terminal,Birdseye Port"
+ },
+ {
+ "name": "HIP 3084",
+ "pos_x": 106.5625,
+ "pos_y": -221.375,
+ "pos_z": 76.15625,
+ "stations": "Hogg Platform,Grego Survey"
+ },
+ {
+ "name": "HIP 30846",
+ "pos_x": 168.78125,
+ "pos_y": -68.25,
+ "pos_z": -72.3125,
+ "stations": "van de Hulst Gateway,Baker Prospect"
+ },
+ {
+ "name": "HIP 30860",
+ "pos_x": -11.625,
+ "pos_y": 36.40625,
+ "pos_z": -154.78125,
+ "stations": "Kneale Platform,Wolfe Beacon"
+ },
+ {
+ "name": "HIP 30944",
+ "pos_x": -3.5,
+ "pos_y": 31.46875,
+ "pos_z": -147.15625,
+ "stations": "Simak Platform"
+ },
+ {
+ "name": "HIP 30953",
+ "pos_x": 150.78125,
+ "pos_y": -67.34375,
+ "pos_z": -29.59375,
+ "stations": "Bigourdan City,Oshima Port,Slipher Enterprise,Sellings Installation,Ingstad Enterprise"
+ },
+ {
+ "name": "HIP 31143",
+ "pos_x": -11.03125,
+ "pos_y": 30.125,
+ "pos_z": -117.125,
+ "stations": "Moore Landing"
+ },
+ {
+ "name": "HIP 31183",
+ "pos_x": -7.78125,
+ "pos_y": 35.75,
+ "pos_z": -149.3125,
+ "stations": "Nylund Outpost,May Sanctuary"
+ },
+ {
+ "name": "HIP 31187",
+ "pos_x": 121.1875,
+ "pos_y": -41.9375,
+ "pos_z": -74.9375,
+ "stations": "Hoyle Port,Hopkinson Vision,Crown Keep,Garrett Vision"
+ },
+ {
+ "name": "HIP 3119",
+ "pos_x": -88.46875,
+ "pos_y": -125.375,
+ "pos_z": -49.8125,
+ "stations": "Thurston Point"
+ },
+ {
+ "name": "HIP 3125",
+ "pos_x": -4.8125,
+ "pos_y": -145.0625,
+ "pos_z": 3.125,
+ "stations": "Glushko Landing,Popper Installation"
+ },
+ {
+ "name": "HIP 31272",
+ "pos_x": -114.875,
+ "pos_y": 83.53125,
+ "pos_z": -132.1875,
+ "stations": "Gessi Depot,Lambert Depot"
+ },
+ {
+ "name": "HIP 31314",
+ "pos_x": 147.5,
+ "pos_y": -81.34375,
+ "pos_z": 51.125,
+ "stations": "Drake Landing,Froud Barracks"
+ },
+ {
+ "name": "HIP 31332",
+ "pos_x": 99.375,
+ "pos_y": -21.96875,
+ "pos_z": -113.9375,
+ "stations": "Henslow Landing,Barcelo Base"
+ },
+ {
+ "name": "HIP 3142",
+ "pos_x": 10.25,
+ "pos_y": -130.0625,
+ "pos_z": 11.75,
+ "stations": "Dyr Terminal,Boss Orbital,Saarinen Installation,Danforth Landing,Zwicky Orbital"
+ },
+ {
+ "name": "HIP 31473",
+ "pos_x": -86.28125,
+ "pos_y": 62.03125,
+ "pos_z": -94.5,
+ "stations": "Byrd Relay,Macomb Vision,Lobachevsky Installation"
+ },
+ {
+ "name": "HIP 3148",
+ "pos_x": 59.25,
+ "pos_y": -210.0625,
+ "pos_z": 45.5625,
+ "stations": "Mainzer Depot"
+ },
+ {
+ "name": "HIP 31509",
+ "pos_x": 111.09375,
+ "pos_y": -40.3125,
+ "pos_z": -52.125,
+ "stations": "Barmin Beacon,Alexander Enterprise,Fu Prospect"
+ },
+ {
+ "name": "HIP 31547",
+ "pos_x": 110.53125,
+ "pos_y": -40,
+ "pos_z": -51.6875,
+ "stations": "Pohl Vision,Sauma Landing"
+ },
+ {
+ "name": "HIP 3161",
+ "pos_x": -78.0625,
+ "pos_y": -126.5625,
+ "pos_z": -43.6875,
+ "stations": "Harrison Terminal,Manarov Plant"
+ },
+ {
+ "name": "HIP 31639",
+ "pos_x": 148.125,
+ "pos_y": -44.53125,
+ "pos_z": -104.9375,
+ "stations": "Fawcett's Folly"
+ },
+ {
+ "name": "HIP 31666",
+ "pos_x": 207.3125,
+ "pos_y": -106.125,
+ "pos_z": 41.09375,
+ "stations": "Shunkai Orbital,Glass Platform,Alexander Landing,Bigourdan Market,Nikitin Installation"
+ },
+ {
+ "name": "HIP 31712",
+ "pos_x": 168.03125,
+ "pos_y": -77.59375,
+ "pos_z": -1.875,
+ "stations": "Reed's Inheritance,Siddha Platform,Salam Vision"
+ },
+ {
+ "name": "HIP 3181",
+ "pos_x": -3.5,
+ "pos_y": -179.21875,
+ "pos_z": 4.75,
+ "stations": "Baade Keep,Iwamoto Gateway,Pilyugin Hub,Agrippa City"
+ },
+ {
+ "name": "HIP 3182",
+ "pos_x": 3.65625,
+ "pos_y": -194.34375,
+ "pos_z": 9.8125,
+ "stations": "Sugie Terminal,Mrkos Vision"
+ },
+ {
+ "name": "HIP 31821",
+ "pos_x": 135.25,
+ "pos_y": -40.3125,
+ "pos_z": -91.21875,
+ "stations": "Humphreys Enterprise,Vespucci Colony,North Settlement"
+ },
+ {
+ "name": "HIP 31833",
+ "pos_x": 129.3125,
+ "pos_y": -53.125,
+ "pos_z": -26.59375,
+ "stations": "Townes City,Rutan Colony,Iqbal Hub"
+ },
+ {
+ "name": "HIP 32001",
+ "pos_x": -59.90625,
+ "pos_y": 58.78125,
+ "pos_z": -125.96875,
+ "stations": "Bresnik Platform,Turtledove Installation"
+ },
+ {
+ "name": "HIP 32103",
+ "pos_x": 162.875,
+ "pos_y": -74.46875,
+ "pos_z": 3,
+ "stations": "Hardy Landing,Shajn Port,Morgan Holdings"
+ },
+ {
+ "name": "HIP 32127",
+ "pos_x": 172.96875,
+ "pos_y": -75.09375,
+ "pos_z": -12.15625,
+ "stations": "Haro Prospect,Bolkow Mine"
+ },
+ {
+ "name": "HIP 32135",
+ "pos_x": -34.96875,
+ "pos_y": 58.96875,
+ "pos_z": -168.625,
+ "stations": "Noli Enterprise,Asimov Bastion"
+ },
+ {
+ "name": "HIP 32242",
+ "pos_x": 151.1875,
+ "pos_y": -63.71875,
+ "pos_z": -15.71875,
+ "stations": "Lewis Relay,Jean Installation"
+ },
+ {
+ "name": "HIP 32350",
+ "pos_x": -10.21875,
+ "pos_y": 50.875,
+ "pos_z": -173.9375,
+ "stations": "Blaylock Reach,Robinson Landing,Bear Settlement"
+ },
+ {
+ "name": "HIP 32351",
+ "pos_x": 212.6875,
+ "pos_y": -121.09375,
+ "pos_z": 99.1875,
+ "stations": "Woolley Terminal,al-Kashi Camp"
+ },
+ {
+ "name": "HIP 32364",
+ "pos_x": 0.96875,
+ "pos_y": 47.21875,
+ "pos_z": -178.34375,
+ "stations": "Lefschetz Settlement,Palmer Settlement,Bloch Vision,Peary Terminal"
+ },
+ {
+ "name": "HIP 32382",
+ "pos_x": -19.84375,
+ "pos_y": 46.25,
+ "pos_z": -139.90625,
+ "stations": "Musabayev Gateway,Morris Gateway"
+ },
+ {
+ "name": "HIP 32435",
+ "pos_x": 145.625,
+ "pos_y": -83.84375,
+ "pos_z": 71.75,
+ "stations": "Nojiri Vision"
+ },
+ {
+ "name": "HIP 32644",
+ "pos_x": 91.78125,
+ "pos_y": -5.3125,
+ "pos_z": -123.71875,
+ "stations": "Pullman Enterprise,Crown Arsenal"
+ },
+ {
+ "name": "HIP 32662",
+ "pos_x": 150.75,
+ "pos_y": -34.59375,
+ "pos_z": -110.28125,
+ "stations": "Holdstock Works,Haber Terminal,Mackenzie Survey"
+ },
+ {
+ "name": "HIP 3267",
+ "pos_x": -128.40625,
+ "pos_y": 8.6875,
+ "pos_z": -81,
+ "stations": "Robinson Camp"
+ },
+ {
+ "name": "HIP 32673",
+ "pos_x": -3.0625,
+ "pos_y": 39.78125,
+ "pos_z": -136.46875,
+ "stations": "Webb Landing,Miller Plant"
+ },
+ {
+ "name": "HIP 32702",
+ "pos_x": -17.75,
+ "pos_y": 46.75,
+ "pos_z": -137.65625,
+ "stations": "Turzillo Works,Margulies Colony,Hawkes Mine"
+ },
+ {
+ "name": "HIP 32728",
+ "pos_x": -0.5,
+ "pos_y": 41.84375,
+ "pos_z": -146.1875,
+ "stations": "Gerrold City,Daley Arsenal"
+ },
+ {
+ "name": "HIP 32806",
+ "pos_x": -48.34375,
+ "pos_y": 50.78125,
+ "pos_z": -104.15625,
+ "stations": "Kiernan Asylum"
+ },
+ {
+ "name": "HIP 32812",
+ "pos_x": 154.65625,
+ "pos_y": -59.4375,
+ "pos_z": -25.1875,
+ "stations": "Kirtley Relay,Froud Hub,Marius Enterprise,Grant Base"
+ },
+ {
+ "name": "HIP 32897",
+ "pos_x": 154.1875,
+ "pos_y": -93.53125,
+ "pos_z": 93.9375,
+ "stations": "Scotti Horizons,Ban Base,MacVicar Hub,Sharp Prospect"
+ },
+ {
+ "name": "HIP 32916",
+ "pos_x": -10.4375,
+ "pos_y": 44.46875,
+ "pos_z": -136.84375,
+ "stations": "Bond Port"
+ },
+ {
+ "name": "HIP 3292",
+ "pos_x": 75.0625,
+ "pos_y": -190.28125,
+ "pos_z": 53.34375,
+ "stations": "Miller Port,Roche Hub,Langley Hub,Heinkel Station,Wood Orbital,Seares Observatory,Otus Gateway,Evans Orbital,Gaensler Gateway,McKee Barracks,Aoki Settlement"
+ },
+ {
+ "name": "HIP 32945",
+ "pos_x": -88.40625,
+ "pos_y": 76.8125,
+ "pos_z": -132.96875,
+ "stations": "McDaniel Installation,Tsunenaga Escape,Valdes Arena"
+ },
+ {
+ "name": "HIP 32970",
+ "pos_x": 131.125,
+ "pos_y": -37.1875,
+ "pos_z": -63.71875,
+ "stations": "Alden Settlement"
+ },
+ {
+ "name": "HIP 33109",
+ "pos_x": 85.40625,
+ "pos_y": -7.4375,
+ "pos_z": -94.875,
+ "stations": "al-Khowarizmi Dock,Cabana Base,Samuda Plant"
+ },
+ {
+ "name": "HIP 3311",
+ "pos_x": -58.28125,
+ "pos_y": -146.6875,
+ "pos_z": -32.125,
+ "stations": "Mohmand Dock,Dzhanibekov Depot"
+ },
+ {
+ "name": "HIP 3318",
+ "pos_x": 50.21875,
+ "pos_y": -190.6875,
+ "pos_z": 37.5,
+ "stations": "Cannon Vision,Hansen Vision,Luyten Plant"
+ },
+ {
+ "name": "HIP 3322",
+ "pos_x": 41.65625,
+ "pos_y": -169.9375,
+ "pos_z": 31.375,
+ "stations": "Penzias Port,McDermott Vision"
+ },
+ {
+ "name": "HIP 3326",
+ "pos_x": -88.9375,
+ "pos_y": -166.65625,
+ "pos_z": -51.0625,
+ "stations": "Kiernan Terminal"
+ },
+ {
+ "name": "HIP 33275",
+ "pos_x": 83.3125,
+ "pos_y": 12.53125,
+ "pos_z": -153.6875,
+ "stations": "Cook Arsenal"
+ },
+ {
+ "name": "HIP 33282",
+ "pos_x": -16.59375,
+ "pos_y": 45.15625,
+ "pos_z": -123.90625,
+ "stations": "Rae Dock,Montrose Dock,d'Eyncourt Dock"
+ },
+ {
+ "name": "HIP 33287",
+ "pos_x": 13.28125,
+ "pos_y": 31.75,
+ "pos_z": -120.75,
+ "stations": "Zelazny City,McDonald Installation,Galindo Ring,Hawke Station,Bulgarin Prospect,Ing Survey"
+ },
+ {
+ "name": "HIP 33368",
+ "pos_x": -29.25,
+ "pos_y": 63.1875,
+ "pos_z": -163.34375,
+ "stations": "Barjavel Station,Resnick Port,Nelson's Folly,Rand Dock,Bokeili Ring,Spectrum Retreat"
+ },
+ {
+ "name": "HIP 33390",
+ "pos_x": 127.375,
+ "pos_y": -52.375,
+ "pos_z": -1.375,
+ "stations": "Opik Port,Grushin Gateway,Rosseland Orbital,Challis Colony,Whelan Ring,Nomen Gateway,Anthony de la Roche Vision,Nikitin Penal colony,Payne-Scott Port,Neujmin Gateway"
+ },
+ {
+ "name": "HIP 33392",
+ "pos_x": 154.6875,
+ "pos_y": -64.875,
+ "pos_z": 2.5625,
+ "stations": "Scotti Stop"
+ },
+ {
+ "name": "HIP 3342",
+ "pos_x": -32.28125,
+ "pos_y": -186.28125,
+ "pos_z": -14.90625,
+ "stations": "Weizsacker Port,Longomontanus Port,Rich Vision,Bachman Keep,Kerr Keep"
+ },
+ {
+ "name": "HIP 3352",
+ "pos_x": 103.40625,
+ "pos_y": -194,
+ "pos_z": 70.90625,
+ "stations": "Hansen Station,Wright Port,Wheeler Dock,Eisinga Enterprise,Fischer Vista"
+ },
+ {
+ "name": "HIP 33536",
+ "pos_x": -75.46875,
+ "pos_y": 83.875,
+ "pos_z": -165.40625,
+ "stations": "Landis Colony,Piccard Base"
+ },
+ {
+ "name": "HIP 33654",
+ "pos_x": 113.96875,
+ "pos_y": -14.71875,
+ "pos_z": -97.1875,
+ "stations": "Alcala Orbital,Quimby City,Hausdorff Prospect,Piper Enterprise,Adams Silo"
+ },
+ {
+ "name": "HIP 33737",
+ "pos_x": 159.875,
+ "pos_y": -85.34375,
+ "pos_z": 62.9375,
+ "stations": "Mueller Vision,Yuzhe Mines,Caselberg Settlement"
+ },
+ {
+ "name": "HIP 33799",
+ "pos_x": 118.25,
+ "pos_y": -19,
+ "pos_z": -86.09375,
+ "stations": "Ellison Port,Siegel Arsenal,Bartlett Station"
+ },
+ {
+ "name": "HIP 33802",
+ "pos_x": 170.21875,
+ "pos_y": -74.8125,
+ "pos_z": 18.96875,
+ "stations": "Antonov Prospect,Payne-Scott Landing,Paola Enterprise"
+ },
+ {
+ "name": "HIP 33818",
+ "pos_x": 43.65625,
+ "pos_y": 22.125,
+ "pos_z": -119.3125,
+ "stations": "Metcalf Vision"
+ },
+ {
+ "name": "HIP 33933",
+ "pos_x": 119.28125,
+ "pos_y": -10.71875,
+ "pos_z": -109.03125,
+ "stations": "Elgin Settlement,Linaweaver Refinery,Ackerman Relay"
+ },
+ {
+ "name": "HIP 34024",
+ "pos_x": -64.53125,
+ "pos_y": 55.40625,
+ "pos_z": -87.3125,
+ "stations": "Moore Installation,Vardeman Holdings"
+ },
+ {
+ "name": "HIP 34212",
+ "pos_x": 165.40625,
+ "pos_y": -77.3125,
+ "pos_z": 36.3125,
+ "stations": "Dubyago Terminal,Hale Settlement,Lockwood's Claim"
+ },
+ {
+ "name": "HIP 34235",
+ "pos_x": -124.78125,
+ "pos_y": 85.6875,
+ "pos_z": -105.46875,
+ "stations": "Hausdorff Relay"
+ },
+ {
+ "name": "HIP 3428",
+ "pos_x": -98.6875,
+ "pos_y": -92.65625,
+ "pos_z": -60.34375,
+ "stations": "Brera Mines"
+ },
+ {
+ "name": "HIP 3434",
+ "pos_x": 28.78125,
+ "pos_y": -206.90625,
+ "pos_z": 23.1875,
+ "stations": "Baliunas Station,Babcock Terminal,Beer Vision,de Kamp Landing,Katzenstein Plant"
+ },
+ {
+ "name": "HIP 34407",
+ "pos_x": 54.03125,
+ "pos_y": 29.5625,
+ "pos_z": -139.5,
+ "stations": "Burgess' Progress,Sladek Vision"
+ },
+ {
+ "name": "HIP 34426",
+ "pos_x": 53.0625,
+ "pos_y": 29.125,
+ "pos_z": -136.84375,
+ "stations": "Adams Prospect,Viehbock Plant"
+ },
+ {
+ "name": "HIP 34540",
+ "pos_x": 54.9375,
+ "pos_y": 33.3125,
+ "pos_z": -147.96875,
+ "stations": "Abasheli Dock"
+ },
+ {
+ "name": "HIP 34698",
+ "pos_x": 127.15625,
+ "pos_y": -46.75,
+ "pos_z": -2.59375,
+ "stations": "Mullane Terminal"
+ },
+ {
+ "name": "HIP 3470",
+ "pos_x": 151.34375,
+ "pos_y": -110.78125,
+ "pos_z": 98.65625,
+ "stations": "Flagg Port,Suzuki Point"
+ },
+ {
+ "name": "HIP 34730",
+ "pos_x": -26.75,
+ "pos_y": 62.84375,
+ "pos_z": -140.90625,
+ "stations": "Newman Camp"
+ },
+ {
+ "name": "HIP 348",
+ "pos_x": -119.53125,
+ "pos_y": -139.5625,
+ "pos_z": -35.53125,
+ "stations": "Hjorth Henriksen"
+ },
+ {
+ "name": "HIP 3488",
+ "pos_x": 152.0625,
+ "pos_y": -190.40625,
+ "pos_z": 100.71875,
+ "stations": "Champlain's Progress,Ballard Installation,Bouwens Oasis,Francis Platform"
+ },
+ {
+ "name": "HIP 34932",
+ "pos_x": 170.71875,
+ "pos_y": -58.15625,
+ "pos_z": -12.375,
+ "stations": "Wilson Base"
+ },
+ {
+ "name": "HIP 34961",
+ "pos_x": 164.78125,
+ "pos_y": -52.25,
+ "pos_z": -21.625,
+ "stations": "Sorayama Station,Maskelyne Dock,Beer Terminal,O'Donnell's Progress,Anderson Relay"
+ },
+ {
+ "name": "HIP 35022",
+ "pos_x": 27.78125,
+ "pos_y": 53.46875,
+ "pos_z": -163.875,
+ "stations": "Egan Asylum,Ing Penal colony"
+ },
+ {
+ "name": "HIP 3509",
+ "pos_x": -124.03125,
+ "pos_y": 11.71875,
+ "pos_z": -79.28125,
+ "stations": "Pryor Port,He Station"
+ },
+ {
+ "name": "HIP 35096",
+ "pos_x": 122.25,
+ "pos_y": -35.59375,
+ "pos_z": -22.65625,
+ "stations": "Morey Orbital,Aleksandrov Plant,Williams Bastion"
+ },
+ {
+ "name": "HIP 35185",
+ "pos_x": 90.40625,
+ "pos_y": 25.4375,
+ "pos_z": -146.96875,
+ "stations": "Brosnan Port,Tayler Works"
+ },
+ {
+ "name": "HIP 35209",
+ "pos_x": 75.8125,
+ "pos_y": 16.40625,
+ "pos_z": -110.28125,
+ "stations": "Coleman City,Locke Gateway,Borlaug Horizons,Forest City,Wiberg Settlement"
+ },
+ {
+ "name": "HIP 35211",
+ "pos_x": 159.21875,
+ "pos_y": -63.1875,
+ "pos_z": 14.59375,
+ "stations": "Corben Platform,Paul Terminal,Chadbourne Colony,Bachman Installation"
+ },
+ {
+ "name": "HIP 35219",
+ "pos_x": 64,
+ "pos_y": 41.40625,
+ "pos_z": -162.3125,
+ "stations": "Wagner Barracks"
+ },
+ {
+ "name": "HIP 35246",
+ "pos_x": 120,
+ "pos_y": -43.34375,
+ "pos_z": 0.4375,
+ "stations": "Extra Station,Perrine Vision,Blaylock Prospect,Wolf Ring"
+ },
+ {
+ "name": "HIP 35274",
+ "pos_x": -58.5,
+ "pos_y": 57.40625,
+ "pos_z": -91.28125,
+ "stations": "Shaw's Exile,Nikitin Mines,Sarrantonio Outpost"
+ },
+ {
+ "name": "HIP 35310",
+ "pos_x": 62.5,
+ "pos_y": 24.90625,
+ "pos_z": -117.90625,
+ "stations": "Ing Beacon"
+ },
+ {
+ "name": "HIP 3540",
+ "pos_x": -53.75,
+ "pos_y": -119.53125,
+ "pos_z": -32.25,
+ "stations": "Watson Hub,Leonov Dock,Malyshev Ring,Midgeley Station,Divis Terminal,Boodt Terminal,Hieb City,Lysenko Orbital,Linnaeus Gateway,Alvarado Prospect,Velho Landing,Robinson Prospect,Otiman Prospect,Stjepan Seljan Point"
+ },
+ {
+ "name": "HIP 35449",
+ "pos_x": -77.90625,
+ "pos_y": 80.96875,
+ "pos_z": -131.25,
+ "stations": "Williamson Settlement,Weyn Prospect,Dashiell Hub,Verne Terminal,McDevitt Terminal"
+ },
+ {
+ "name": "HIP 3551",
+ "pos_x": -97.96875,
+ "pos_y": -117.65625,
+ "pos_z": -60.625,
+ "stations": "Sawyer Stop,Simmons Landing"
+ },
+ {
+ "name": "HIP 35531",
+ "pos_x": 162.3125,
+ "pos_y": -48.71875,
+ "pos_z": -20.25,
+ "stations": "King Dock,Schroeder Orbital"
+ },
+ {
+ "name": "HIP 35661",
+ "pos_x": 205.9375,
+ "pos_y": -106.1875,
+ "pos_z": 83.09375,
+ "stations": "Shoujing Beacon,Elbakyan Mine,Leinster Base"
+ },
+ {
+ "name": "HIP 35718",
+ "pos_x": 94.75,
+ "pos_y": 17.28125,
+ "pos_z": -119.15625,
+ "stations": "Waldeck Base,Erikson Mines,Lee Bastion"
+ },
+ {
+ "name": "HIP 35755",
+ "pos_x": 5.5625,
+ "pos_y": 46.03125,
+ "pos_z": -113.8125,
+ "stations": "Arkwright Hub,Maxwell Ring,Nagel Refinery,Robinson Vision,Shumil Installation"
+ },
+ {
+ "name": "HIP 35803",
+ "pos_x": 44.8125,
+ "pos_y": 36.3125,
+ "pos_z": -122,
+ "stations": "Kovalevsky Gateway,Torricelli City,Guest Port,Kwolek Station,Bounds Hub,Block Relay,Ogden Installation,Vela Gateway"
+ },
+ {
+ "name": "HIP 3587",
+ "pos_x": -133.875,
+ "pos_y": 10.8125,
+ "pos_z": -85.90625,
+ "stations": "Virtanen Depot,Porsche Depot,Kettle's Progress"
+ },
+ {
+ "name": "HIP 35873",
+ "pos_x": 63.1875,
+ "pos_y": 30.65625,
+ "pos_z": -122.34375,
+ "stations": "Pook Dock,Bendell Dock,Gardner Relay,Haldeman Horizons"
+ },
+ {
+ "name": "HIP 35910",
+ "pos_x": 157,
+ "pos_y": -53.3125,
+ "pos_z": -0.15625,
+ "stations": "Zubrin Dock,Hickman Station,Artsutanov Landing,Hume Barracks,Struve Dock"
+ },
+ {
+ "name": "HIP 35965",
+ "pos_x": 170.375,
+ "pos_y": -48.71875,
+ "pos_z": -20.6875,
+ "stations": "Brash Dock,Duffy Plant,Love Hub,Foale Hub,Terry Hub"
+ },
+ {
+ "name": "HIP 35992",
+ "pos_x": 143.875,
+ "pos_y": -27.3125,
+ "pos_z": -49.28125,
+ "stations": "Brongniart Enterprise,Hale Dock,Anthony Base,Elion Port,Al-Farabi Depot"
+ },
+ {
+ "name": "HIP 36014",
+ "pos_x": -40.84375,
+ "pos_y": 64.5625,
+ "pos_z": -117.375,
+ "stations": "Cenker Base,Broglie Platform"
+ },
+ {
+ "name": "HIP 3603",
+ "pos_x": 43.125,
+ "pos_y": -175.03125,
+ "pos_z": 29.96875,
+ "stations": "Guth Station,Nicholson Barracks"
+ },
+ {
+ "name": "HIP 36037",
+ "pos_x": -11.03125,
+ "pos_y": 53.125,
+ "pos_z": -113.8125,
+ "stations": "Lyakhov Terminal,Ray Mines,Agassiz Terminal,Fox Prospect"
+ },
+ {
+ "name": "HIP 36081",
+ "pos_x": 112.1875,
+ "pos_y": 1.90625,
+ "pos_z": -90.46875,
+ "stations": "Baird Ring,Fidalgo's Inheritance,Betancourt Vision,Wallis Orbital,Peral Prospect"
+ },
+ {
+ "name": "HIP 36129",
+ "pos_x": -45.34375,
+ "pos_y": 60.5625,
+ "pos_z": -103.71875,
+ "stations": "Palmer Port,Rasmussen Survey"
+ },
+ {
+ "name": "HIP 36160",
+ "pos_x": 114.375,
+ "pos_y": -17.3125,
+ "pos_z": -47.03125,
+ "stations": "Bradbury Reformatory,Wolfe Dock"
+ },
+ {
+ "name": "HIP 36165",
+ "pos_x": 118.5,
+ "pos_y": -17.9375,
+ "pos_z": -48.71875,
+ "stations": "Nakano Mines,Taylor Jr. Landing,Clebsch Survey"
+ },
+ {
+ "name": "HIP 36198",
+ "pos_x": 172.4375,
+ "pos_y": -68.375,
+ "pos_z": 25.40625,
+ "stations": "Thomson Outpost"
+ },
+ {
+ "name": "HIP 3629",
+ "pos_x": 2.625,
+ "pos_y": -225.21875,
+ "pos_z": 4.25,
+ "stations": "Eggleton Port,Merritt Orbital,Wilder Beacon"
+ },
+ {
+ "name": "HIP 3633",
+ "pos_x": -90.5,
+ "pos_y": -119.8125,
+ "pos_z": -56.8125,
+ "stations": "Minkowski Landing"
+ },
+ {
+ "name": "HIP 36352",
+ "pos_x": 119.0625,
+ "pos_y": -38.90625,
+ "pos_z": -0.0625,
+ "stations": "Chadwick Mines,Iwamoto Vision"
+ },
+ {
+ "name": "HIP 3643",
+ "pos_x": 22.3125,
+ "pos_y": -181.875,
+ "pos_z": 16.3125,
+ "stations": "Kidd Terminal"
+ },
+ {
+ "name": "HIP 36485",
+ "pos_x": 60.59375,
+ "pos_y": 39.28125,
+ "pos_z": -129.34375,
+ "stations": "Alexeyev Hub,Fermat Survey,Hartog Arsenal"
+ },
+ {
+ "name": "HIP 36509",
+ "pos_x": 31.84375,
+ "pos_y": 61.03125,
+ "pos_z": -156.375,
+ "stations": "Cochrane Barracks,Forbes Horizons"
+ },
+ {
+ "name": "HIP 36512",
+ "pos_x": 94.875,
+ "pos_y": 3.3125,
+ "pos_z": -74.28125,
+ "stations": "Wallis Camp,Hoyle Bastion"
+ },
+ {
+ "name": "HIP 36560",
+ "pos_x": 43.625,
+ "pos_y": 54.9375,
+ "pos_z": -150.4375,
+ "stations": "Rothman Horizons,Rawn Installation"
+ },
+ {
+ "name": "HIP 36622",
+ "pos_x": 148.1875,
+ "pos_y": -51.375,
+ "pos_z": 8.9375,
+ "stations": "Fowler Vision,Sucharitkul Installation,Benford Enterprise"
+ },
+ {
+ "name": "HIP 36731",
+ "pos_x": 125.8125,
+ "pos_y": -2.53125,
+ "pos_z": -79.40625,
+ "stations": "Hire Base"
+ },
+ {
+ "name": "HIP 36866",
+ "pos_x": 130.21875,
+ "pos_y": -49.65625,
+ "pos_z": 19.375,
+ "stations": "Mieville Depot,Bus Platform,Baillaud Platform"
+ },
+ {
+ "name": "HIP 36954",
+ "pos_x": -66.59375,
+ "pos_y": 61.0625,
+ "pos_z": -84.90625,
+ "stations": "Parry Enterprise,Stephens Ring,Yano Station,Moore Survey,Kelly Depot"
+ },
+ {
+ "name": "HIP 36964",
+ "pos_x": 142.3125,
+ "pos_y": -15.1875,
+ "pos_z": -59.71875,
+ "stations": "Fernao do Po Dock,Malzberg's Claim"
+ },
+ {
+ "name": "HIP 37162",
+ "pos_x": -8.9375,
+ "pos_y": 59.5,
+ "pos_z": -116.375,
+ "stations": "Serebrov Ring,Clifford's Folly,Knight Beacon,Crippen Terminal"
+ },
+ {
+ "name": "HIP 37170",
+ "pos_x": -17.71875,
+ "pos_y": 60,
+ "pos_z": -111.9375,
+ "stations": "Crown Orbital"
+ },
+ {
+ "name": "HIP 37171",
+ "pos_x": 145.0625,
+ "pos_y": -6.78125,
+ "pos_z": -75.4375,
+ "stations": "Burnham Freeport"
+ },
+ {
+ "name": "HIP 3724",
+ "pos_x": -78.46875,
+ "pos_y": -140.84375,
+ "pos_z": -49.90625,
+ "stations": "de Andrade Depot,Rondon Penal colony"
+ },
+ {
+ "name": "HIP 37376",
+ "pos_x": 82.15625,
+ "pos_y": 53.25,
+ "pos_z": -155.75,
+ "stations": "Kippax Stop"
+ },
+ {
+ "name": "HIP 37520",
+ "pos_x": 128.34375,
+ "pos_y": 17.71875,
+ "pos_z": -108.90625,
+ "stations": "Grover Terminal"
+ },
+ {
+ "name": "HIP 37645",
+ "pos_x": 95.34375,
+ "pos_y": 39.34375,
+ "pos_z": -130.28125,
+ "stations": "Chebyshev Dock,Archer Enterprise,Rice Landing,Piserchia Bastion"
+ },
+ {
+ "name": "HIP 37683",
+ "pos_x": 167.25,
+ "pos_y": -33.15625,
+ "pos_z": -28.3125,
+ "stations": "Rigaux Stop"
+ },
+ {
+ "name": "HIP 37722",
+ "pos_x": 55.15625,
+ "pos_y": 60.59375,
+ "pos_z": -148.3125,
+ "stations": "Rusch Landing,Harding Settlement"
+ },
+ {
+ "name": "HIP 37735",
+ "pos_x": 137.125,
+ "pos_y": -53.5,
+ "pos_z": 28.3125,
+ "stations": "Kanai Colony,Bernoulli Vision,Hay Enterprise"
+ },
+ {
+ "name": "HIP 37844",
+ "pos_x": 134.5625,
+ "pos_y": 23.875,
+ "pos_z": -118.53125,
+ "stations": "Drummond Vision"
+ },
+ {
+ "name": "HIP 3788",
+ "pos_x": 3.09375,
+ "pos_y": -201.9375,
+ "pos_z": 2.28125,
+ "stations": "Zarnecki Terminal,de Kamp Relay"
+ },
+ {
+ "name": "HIP 37918",
+ "pos_x": 121.28125,
+ "pos_y": -36.46875,
+ "pos_z": 5.125,
+ "stations": "Sucharitkul Keep,Schiaparelli Hangar,Charlois Settlement,Gasparis Dock"
+ },
+ {
+ "name": "HIP 38129",
+ "pos_x": 127.125,
+ "pos_y": -13.96875,
+ "pos_z": -38.3125,
+ "stations": "Blodgett Port,Titov Hub,Thorne Ring,Culpeper Market,Warner Base,Curie Dock,Coulomb Horizons,Skvortsov Gateway,Parmitano Dock,Scheerbart Base,Galvani Orbital,Lem Prospect,Becquerel Ring,King Mines,Tenn Horizons"
+ },
+ {
+ "name": "HIP 38134",
+ "pos_x": 144.34375,
+ "pos_y": 2.21875,
+ "pos_z": -77.25,
+ "stations": "Pinto Vision,Wagner Station,Russell Barracks,Verrazzano Camp"
+ },
+ {
+ "name": "HIP 38172",
+ "pos_x": 36.71875,
+ "pos_y": 56.34375,
+ "pos_z": -123.40625,
+ "stations": "Hurley Landing,Leestma Camp"
+ },
+ {
+ "name": "HIP 38225",
+ "pos_x": -103.875,
+ "pos_y": 69.96875,
+ "pos_z": -78.21875,
+ "stations": "Plexico City,Gloss Station,Lewis Gateway,Lupoff Landing"
+ },
+ {
+ "name": "HIP 38235",
+ "pos_x": 174.34375,
+ "pos_y": 5.5625,
+ "pos_z": -96.75,
+ "stations": "Pytheas Dock,Rae Port,Mille Survey,Rothman's Pride,Kimberlin Penal colony"
+ },
+ {
+ "name": "HIP 38422",
+ "pos_x": 8.5,
+ "pos_y": 59.65625,
+ "pos_z": -112.59375,
+ "stations": "Larbalestier Hub,Hinz Arsenal"
+ },
+ {
+ "name": "HIP 38531",
+ "pos_x": 156.21875,
+ "pos_y": -11.21875,
+ "pos_z": -52.34375,
+ "stations": "Dyr Terminal,Hodgson Port,Auld Prospect"
+ },
+ {
+ "name": "HIP 3864",
+ "pos_x": 104.0625,
+ "pos_y": -245.5625,
+ "pos_z": 67.09375,
+ "stations": "Luu Orbital,Weaver Orbital,Nilson Enterprise"
+ },
+ {
+ "name": "HIP 38646",
+ "pos_x": 154.125,
+ "pos_y": -29.9375,
+ "pos_z": -16.34375,
+ "stations": "Erikson Vision,Giles Platform,Macgregor Terminal,Vyssotsky Terminal"
+ },
+ {
+ "name": "HIP 38747",
+ "pos_x": 111.71875,
+ "pos_y": 25.84375,
+ "pos_z": -95.03125,
+ "stations": "Smith Landing,Hodgson Terminal,Cayley Settlement,Boucher Horizons,Taine Laboratory"
+ },
+ {
+ "name": "HIP 38862",
+ "pos_x": 113.96875,
+ "pos_y": 19.34375,
+ "pos_z": -82.96875,
+ "stations": "Eschbach Enterprise,Barcelo Enterprise,Gottlob Frege Terminal"
+ },
+ {
+ "name": "HIP 39088",
+ "pos_x": 157,
+ "pos_y": -41.84375,
+ "pos_z": 7.375,
+ "stations": "Cowell Platform,Shipton Relay,Bohr Terminal"
+ },
+ {
+ "name": "HIP 3911",
+ "pos_x": 133.65625,
+ "pos_y": -106.09375,
+ "pos_z": 86.65625,
+ "stations": "Giclas Orbital,Panshin Survey"
+ },
+ {
+ "name": "HIP 39242",
+ "pos_x": 161.40625,
+ "pos_y": -59.5,
+ "pos_z": 36.71875,
+ "stations": "Tyson Terminal,Bode Hub,Hansen Port,Young Arsenal,Macan Survey"
+ },
+ {
+ "name": "HIP 3930",
+ "pos_x": -127.75,
+ "pos_y": -8.28125,
+ "pos_z": -83.5625,
+ "stations": "Pond City,Houtman Settlement,Pratchett Gateway,McCormick Town,Verne's Inheritance"
+ },
+ {
+ "name": "HIP 39313",
+ "pos_x": -64.46875,
+ "pos_y": 101.4375,
+ "pos_z": -145.40625,
+ "stations": "Ivens Dock,Woolley Terminal,Starzl Dock,Frobisher Installation,MacCurdy Settlement"
+ },
+ {
+ "name": "HIP 39325",
+ "pos_x": 77.09375,
+ "pos_y": 41.28125,
+ "pos_z": -99.25,
+ "stations": "Dyomin Prospect"
+ },
+ {
+ "name": "HIP 39330",
+ "pos_x": 118.625,
+ "pos_y": -16.9375,
+ "pos_z": -17.59375,
+ "stations": "Gonnessiat Landing"
+ },
+ {
+ "name": "HIP 39391",
+ "pos_x": 157.5,
+ "pos_y": -33.28125,
+ "pos_z": -4.6875,
+ "stations": "Neville Platform,Lenthall Dock,Goulart Dock"
+ },
+ {
+ "name": "HIP 39417",
+ "pos_x": 117.1875,
+ "pos_y": 49.0625,
+ "pos_z": -126.25,
+ "stations": "Avdeyev Stop"
+ },
+ {
+ "name": "HIP 39418",
+ "pos_x": 67.1875,
+ "pos_y": 53.71875,
+ "pos_z": -115,
+ "stations": "Lovell Platform,Wallace Terminal,Montrose Relay"
+ },
+ {
+ "name": "HIP 39470",
+ "pos_x": 133.9375,
+ "pos_y": -4.625,
+ "pos_z": -42.65625,
+ "stations": "Song Orbital,Wang Settlement,Martinez Vision"
+ },
+ {
+ "name": "HIP 39515",
+ "pos_x": 54.125,
+ "pos_y": 51.4375,
+ "pos_z": -105.03125,
+ "stations": "Rothman Installation"
+ },
+ {
+ "name": "HIP 39528",
+ "pos_x": 41.1875,
+ "pos_y": 67.28125,
+ "pos_z": -126.25,
+ "stations": "Henderson Survey,Nansen Vision"
+ },
+ {
+ "name": "HIP 39546",
+ "pos_x": 36.65625,
+ "pos_y": 57.03125,
+ "pos_z": -107.53125,
+ "stations": "Bushkov Base,Bates Landing"
+ },
+ {
+ "name": "HIP 39681",
+ "pos_x": 61.46875,
+ "pos_y": 89.25,
+ "pos_z": -167.0625,
+ "stations": "Crown's Exile"
+ },
+ {
+ "name": "HIP 39720",
+ "pos_x": 147.21875,
+ "pos_y": -5.21875,
+ "pos_z": -43.3125,
+ "stations": "Lawrence Station,Farrer Terminal,Stevin Beacon"
+ },
+ {
+ "name": "HIP 39821",
+ "pos_x": 70.96875,
+ "pos_y": 50.875,
+ "pos_z": -106.21875,
+ "stations": "Vinge Settlement,Shiner Platform,Butcher Horizons"
+ },
+ {
+ "name": "HIP 39887",
+ "pos_x": 140.34375,
+ "pos_y": -60.71875,
+ "pos_z": 49.5,
+ "stations": "Pimi Ring,Volterra Terminal,Rosse Arsenal"
+ },
+ {
+ "name": "HIP 39908",
+ "pos_x": 131.9375,
+ "pos_y": 18.84375,
+ "pos_z": -74.625,
+ "stations": "Hobaugh Vision,Kuttner Depot,Hoard Settlement,Steiner Beacon"
+ },
+ {
+ "name": "HIP 3999",
+ "pos_x": 27.78125,
+ "pos_y": -164.03125,
+ "pos_z": 16.4375,
+ "stations": "Wisdom Landing,Hevelius' Progress,Slipher Mines,Sellings Prospect,Ohain Hub"
+ },
+ {
+ "name": "HIP 4003",
+ "pos_x": 121.65625,
+ "pos_y": -116.28125,
+ "pos_z": 78.5625,
+ "stations": "Ptack Hub"
+ },
+ {
+ "name": "HIP 4005",
+ "pos_x": -125.53125,
+ "pos_y": -86.78125,
+ "pos_z": -83.375,
+ "stations": "Chebyshev Dock,Thurston Dock,Rochon Mines,Sucharitkul Landing"
+ },
+ {
+ "name": "HIP 40140",
+ "pos_x": 21.0625,
+ "pos_y": 69.125,
+ "pos_z": -114.8125,
+ "stations": "Dietz Dock,Alexandria City,Toll Orbital"
+ },
+ {
+ "name": "HIP 40170",
+ "pos_x": -15.625,
+ "pos_y": 46.34375,
+ "pos_z": -67.375,
+ "stations": "Sanger Station,Bridges Dock,Bixby Depot"
+ },
+ {
+ "name": "HIP 40174",
+ "pos_x": 133.375,
+ "pos_y": -8.09375,
+ "pos_z": -29.34375,
+ "stations": "Clauss Relay,Birmingham Outpost,Somerset Vista"
+ },
+ {
+ "name": "HIP 4024",
+ "pos_x": -22.09375,
+ "pos_y": -122.65625,
+ "pos_z": -15.96875,
+ "stations": "Leberecht Tempel Hub,Gurevich City,Hassanein Keep,Andersson Beacon"
+ },
+ {
+ "name": "HIP 40420",
+ "pos_x": 59.34375,
+ "pos_y": 59.5,
+ "pos_z": -108.34375,
+ "stations": "Constantine Terminal,Abnett Port,Lloyd Port,Santos Station,Malcolm Market,von Krusenstern Terminal,Arnason Port,la Cosa Port,Antonio de Andrade Palace,Jiushao Port,Stanley Depot"
+ },
+ {
+ "name": "HIP 40449",
+ "pos_x": 79.3125,
+ "pos_y": 62.5625,
+ "pos_z": -118.375,
+ "stations": "Deb Plant,Stafford Settlement"
+ },
+ {
+ "name": "HIP 4045",
+ "pos_x": 50.125,
+ "pos_y": -253.9375,
+ "pos_z": 29.59375,
+ "stations": "Dawes Terminal,Sitterly Mines,Thomson Survey,Pollas Arsenal,Markham Vision"
+ },
+ {
+ "name": "HIP 40452",
+ "pos_x": 61.375,
+ "pos_y": 51.8125,
+ "pos_z": -96.78125,
+ "stations": "Carlisle Terminal,Geston Ring,Tudela Terminal,Paulo da Gama Terminal,Derleth Port"
+ },
+ {
+ "name": "HIP 40465",
+ "pos_x": 103.90625,
+ "pos_y": 32.15625,
+ "pos_z": -79,
+ "stations": "Leopold Holdings,Garay Colony,Lloyd Depot"
+ },
+ {
+ "name": "HIP 40527",
+ "pos_x": -90.125,
+ "pos_y": 75.25,
+ "pos_z": -88.125,
+ "stations": "Dias Arsenal,Marshall Installation"
+ },
+ {
+ "name": "HIP 4062",
+ "pos_x": 171.625,
+ "pos_y": -187.1875,
+ "pos_z": 110.3125,
+ "stations": "Brothers Platform,Goodricke Platform,Baliunas Colony,Ayton Innes Beacon,Resnick Base"
+ },
+ {
+ "name": "HIP 4072",
+ "pos_x": -10.875,
+ "pos_y": -172.15625,
+ "pos_z": -9.71875,
+ "stations": "Kotelnikov Hangar,Russell's Pride,Smoot Camp"
+ },
+ {
+ "name": "HIP 4073",
+ "pos_x": -21.71875,
+ "pos_y": -254.46875,
+ "pos_z": -18.09375,
+ "stations": "Herbig Settlement,Somerville Platform,Kranz Vision"
+ },
+ {
+ "name": "HIP 40732",
+ "pos_x": 111.375,
+ "pos_y": 50.03125,
+ "pos_z": -104.4375,
+ "stations": "Efremov Horizons,Alvares Settlement,Crowley Park,Lee Lab"
+ },
+ {
+ "name": "HIP 40786",
+ "pos_x": 145.5,
+ "pos_y": 44.0625,
+ "pos_z": -103.75,
+ "stations": "Bunch Enterprise,Goonan Base"
+ },
+ {
+ "name": "HIP 40928",
+ "pos_x": 2.75,
+ "pos_y": 103.4375,
+ "pos_z": -151.96875,
+ "stations": "Kozeyev's Progress,Haberlandt Oasis"
+ },
+ {
+ "name": "HIP 40942",
+ "pos_x": 16.25,
+ "pos_y": 74.65625,
+ "pos_z": -113.15625,
+ "stations": "Dana Colony,Adams Enterprise,Baird Installation"
+ },
+ {
+ "name": "HIP 40977",
+ "pos_x": 49.3125,
+ "pos_y": 57.71875,
+ "pos_z": -96.40625,
+ "stations": "White Port"
+ },
+ {
+ "name": "HIP 41022",
+ "pos_x": -48.9375,
+ "pos_y": 106.15625,
+ "pos_z": -141.90625,
+ "stations": "Neff Terminal,Ford Platform"
+ },
+ {
+ "name": "HIP 4103",
+ "pos_x": -89.5625,
+ "pos_y": -65.21875,
+ "pos_z": -60.21875,
+ "stations": "Utley Dock,Bellamy Dock"
+ },
+ {
+ "name": "HIP 41088",
+ "pos_x": 94.9375,
+ "pos_y": 71.25,
+ "pos_z": -125.90625,
+ "stations": "Morgan Bastion"
+ },
+ {
+ "name": "HIP 41090",
+ "pos_x": 74.3125,
+ "pos_y": 55.78125,
+ "pos_z": -98.5625,
+ "stations": "Henderson City,Palmer Dock,Miletus Terminal,Gunn Base"
+ },
+ {
+ "name": "HIP 41181",
+ "pos_x": -8.15625,
+ "pos_y": 74.8125,
+ "pos_z": -105.125,
+ "stations": "Andersson Station,Stevens Barracks,Garcia Port"
+ },
+ {
+ "name": "HIP 41184",
+ "pos_x": -7.78125,
+ "pos_y": 71.25,
+ "pos_z": -100.125,
+ "stations": "Asire Market,Anderson Lab,Carlisle Barracks,Lee Station,Turtledove Enterprise"
+ },
+ {
+ "name": "HIP 4120",
+ "pos_x": -77.6875,
+ "pos_y": -124.1875,
+ "pos_z": -53.46875,
+ "stations": "Jeury Dock,Wylie Dock"
+ },
+ {
+ "name": "HIP 41308",
+ "pos_x": -51,
+ "pos_y": 68.625,
+ "pos_z": -85.71875,
+ "stations": "Panshin Orbital,Andrews Terminal,Burkin Terminal,Smith Hub,Verrazzano Terminal,Hughes Hub,Galindo City,Reed Dock,Qurra Vision,Kinsey Enterprise,Bachman Horizons,Diamond Bastion,Hendel Terminal,Francisco de Eliza Port,Saberhagen Laboratory,Godwin Settlement"
+ },
+ {
+ "name": "HIP 41322",
+ "pos_x": 125.25,
+ "pos_y": 32.78125,
+ "pos_z": -74.5625,
+ "stations": "Macarthur Colony,Carpenter Port"
+ },
+ {
+ "name": "HIP 41360",
+ "pos_x": 160.15625,
+ "pos_y": 20.03125,
+ "pos_z": -63.8125,
+ "stations": "Grant Vision,Bogdanov Port"
+ },
+ {
+ "name": "HIP 41426",
+ "pos_x": 174.46875,
+ "pos_y": -25.25,
+ "pos_z": -2.625,
+ "stations": "Horch Port,Schrodinger Beacon,Bishop's Progress"
+ },
+ {
+ "name": "HIP 41479",
+ "pos_x": 94.09375,
+ "pos_y": 42.78125,
+ "pos_z": -79.9375,
+ "stations": "Fibonacci Refinery,Atwood Survey"
+ },
+ {
+ "name": "HIP 41503",
+ "pos_x": 129.375,
+ "pos_y": 30.71875,
+ "pos_z": -70.34375,
+ "stations": "Lorentz Base,Goeschke Outpost,Grothendieck Beacon"
+ },
+ {
+ "name": "HIP 41526",
+ "pos_x": -14.53125,
+ "pos_y": 83.875,
+ "pos_z": -113.71875,
+ "stations": "Gernsback Hangar,Barnes Beacon"
+ },
+ {
+ "name": "HIP 41529",
+ "pos_x": 129.40625,
+ "pos_y": 3.09375,
+ "pos_z": -31.5625,
+ "stations": "Sargent Port,Tepper City,Fremont Relay"
+ },
+ {
+ "name": "HIP 41573",
+ "pos_x": 93.40625,
+ "pos_y": 56,
+ "pos_z": -97.15625,
+ "stations": "Pelliot Terminal,Beliaev Landing,Weitz Bastion"
+ },
+ {
+ "name": "HIP 41637",
+ "pos_x": -40.21875,
+ "pos_y": 101.34375,
+ "pos_z": -131.65625,
+ "stations": "von Helmont Survey"
+ },
+ {
+ "name": "HIP 41659",
+ "pos_x": 165.78125,
+ "pos_y": -25.71875,
+ "pos_z": 1.9375,
+ "stations": "Pollas Port"
+ },
+ {
+ "name": "HIP 41681",
+ "pos_x": 159.40625,
+ "pos_y": -12.65625,
+ "pos_z": -14.65625,
+ "stations": "Griffith Mines"
+ },
+ {
+ "name": "HIP 41810",
+ "pos_x": -34.9375,
+ "pos_y": 93.40625,
+ "pos_z": -120.40625,
+ "stations": "Salgari Hub"
+ },
+ {
+ "name": "HIP 41844",
+ "pos_x": -21.28125,
+ "pos_y": 80.8125,
+ "pos_z": -105.59375,
+ "stations": "Dietz Vision,Nylund Port,Goonan Installation"
+ },
+ {
+ "name": "HIP 41955",
+ "pos_x": -28.875,
+ "pos_y": 72.6875,
+ "pos_z": -92.53125,
+ "stations": "Nixon Landing,Lukyanenko Platform,Fibonacci Refinery"
+ },
+ {
+ "name": "HIP 41967",
+ "pos_x": 138.53125,
+ "pos_y": 15.875,
+ "pos_z": -46.4375,
+ "stations": "Jacobi Prospect,Alvares Keep,Alexandrov Hangar"
+ },
+ {
+ "name": "HIP 41968",
+ "pos_x": 137.71875,
+ "pos_y": 65.65625,
+ "pos_z": -113.125,
+ "stations": "Yano's Claim"
+ },
+ {
+ "name": "HIP 41984",
+ "pos_x": 150.375,
+ "pos_y": -2.0625,
+ "pos_z": -24.28125,
+ "stations": "Clark Station,McCandless Horizons,Crumey Penal colony"
+ },
+ {
+ "name": "HIP 42042",
+ "pos_x": 143.4375,
+ "pos_y": 5.59375,
+ "pos_z": -32.71875,
+ "stations": "Felice Camp,Baraniecki Survey,Bosch Base"
+ },
+ {
+ "name": "HIP 42174",
+ "pos_x": 149.15625,
+ "pos_y": 46.78125,
+ "pos_z": -86.59375,
+ "stations": "Fontana Platform"
+ },
+ {
+ "name": "HIP 42196",
+ "pos_x": 125.90625,
+ "pos_y": -0.75,
+ "pos_z": -19.75,
+ "stations": "Mukai Dock"
+ },
+ {
+ "name": "HIP 42214",
+ "pos_x": 116.28125,
+ "pos_y": 14.375,
+ "pos_z": -37.9375,
+ "stations": "Hartsfield Settlement,Cunningham Arsenal"
+ },
+ {
+ "name": "HIP 42253",
+ "pos_x": 44.9375,
+ "pos_y": 79.25,
+ "pos_z": -111.09375,
+ "stations": "Phillpotts Port,Steele Ring,Ashton Vision,Needham's Inheritance,Ford Arsenal"
+ },
+ {
+ "name": "HIP 42279",
+ "pos_x": -83.34375,
+ "pos_y": 77.75,
+ "pos_z": -88.46875,
+ "stations": "Sagan Enterprise,Loncke Keep,Oppenheimer Vision"
+ },
+ {
+ "name": "HIP 42371",
+ "pos_x": 26.25,
+ "pos_y": 74.0625,
+ "pos_z": -100.15625,
+ "stations": "Hopper Beacon,Koch Settlement,Morrow Survey"
+ },
+ {
+ "name": "HIP 42408",
+ "pos_x": 99.40625,
+ "pos_y": 48.625,
+ "pos_z": -77.8125,
+ "stations": "Hilmers Works"
+ },
+ {
+ "name": "HIP 4242",
+ "pos_x": -25.1875,
+ "pos_y": -199,
+ "pos_z": -21.5,
+ "stations": "Niemeyer Terminal,Wenzel Prospect,Soto Survey,Hague Terminal"
+ },
+ {
+ "name": "HIP 42455",
+ "pos_x": 127.90625,
+ "pos_y": 7.28125,
+ "pos_z": -28.25,
+ "stations": "Kingsbury Terminal,Aguirre Gateway,Mouhot Depot,Tarski Prospect,Barnes Base"
+ },
+ {
+ "name": "HIP 42532",
+ "pos_x": 147.34375,
+ "pos_y": -49.78125,
+ "pos_z": 42.78125,
+ "stations": "Gotz Ring,Wilson Dock"
+ },
+ {
+ "name": "HIP 42539",
+ "pos_x": 147.40625,
+ "pos_y": -65.5625,
+ "pos_z": 63,
+ "stations": "Folland Prospect,Bottego Base,Anderson Terminal"
+ },
+ {
+ "name": "HIP 42563",
+ "pos_x": 80.5,
+ "pos_y": 87.8125,
+ "pos_z": -123.59375,
+ "stations": "Weitz Hub,Budarin Gateway"
+ },
+ {
+ "name": "HIP 4261",
+ "pos_x": 80.8125,
+ "pos_y": -237.53125,
+ "pos_z": 47.78125,
+ "stations": "Charlier Platform,Moffat Relay,Collins Base"
+ },
+ {
+ "name": "HIP 42695",
+ "pos_x": 161.625,
+ "pos_y": -18.21875,
+ "pos_z": 1.59375,
+ "stations": "Buckell Enterprise"
+ },
+ {
+ "name": "HIP 42727",
+ "pos_x": 174.25,
+ "pos_y": -56.6875,
+ "pos_z": 48.75,
+ "stations": "Marriott Orbital,LeConte Terminal"
+ },
+ {
+ "name": "HIP 4273",
+ "pos_x": 149.53125,
+ "pos_y": -225.25,
+ "pos_z": 93.59375,
+ "stations": "Hewish Landing,van den Hove Landing"
+ },
+ {
+ "name": "HIP 42783",
+ "pos_x": 36.125,
+ "pos_y": 69.125,
+ "pos_z": -91.5625,
+ "stations": "Barratt Dock,Romanenko Orbital,Smith Station,Glazkov Station,Glidden Orbital,Crown Forum,Lee Depot,Arthur's Claim,Robinson Dock,Fisher City"
+ },
+ {
+ "name": "HIP 42885",
+ "pos_x": 165.1875,
+ "pos_y": -27.03125,
+ "pos_z": 13.71875,
+ "stations": "Sugie Survey,Nachtigal Relay"
+ },
+ {
+ "name": "HIP 4291",
+ "pos_x": 102.21875,
+ "pos_y": -113.34375,
+ "pos_z": 64.96875,
+ "stations": "Fukushima Dock"
+ },
+ {
+ "name": "HIP 42951",
+ "pos_x": 140.34375,
+ "pos_y": 82.625,
+ "pos_z": -118.5625,
+ "stations": "Serre Depot"
+ },
+ {
+ "name": "HIP 43054",
+ "pos_x": 149.59375,
+ "pos_y": 41.84375,
+ "pos_z": -68.09375,
+ "stations": "Hoshi Refinery,Compton City,Darlton Installation"
+ },
+ {
+ "name": "HIP 4310",
+ "pos_x": -21.96875,
+ "pos_y": -156.96875,
+ "pos_z": -19.21875,
+ "stations": "Herzog Station,Crown Depot,Amedeo Plana Orbital,Chawla Holdings"
+ },
+ {
+ "name": "HIP 4312",
+ "pos_x": -53.84375,
+ "pos_y": -123.59375,
+ "pos_z": -39.5,
+ "stations": "Eratosthenes Camp"
+ },
+ {
+ "name": "HIP 43197",
+ "pos_x": 139.875,
+ "pos_y": -9.0625,
+ "pos_z": -3.28125,
+ "stations": "Goldstein Base,White Terminal,Vonnegut Port,Daniel Camp"
+ },
+ {
+ "name": "HIP 43296",
+ "pos_x": 11.84375,
+ "pos_y": 82.28125,
+ "pos_z": -100.46875,
+ "stations": "Ito Station,Jahn Hub,Nusslein-Volhard Ring,Hoyle Observatory,Barry Gateway,Galton Port,Prunariu Point,Hume's Claim"
+ },
+ {
+ "name": "HIP 43299",
+ "pos_x": 109.625,
+ "pos_y": 39.78125,
+ "pos_z": -58.5625,
+ "stations": "Malenchenko Survey,Guidoni Station,Kennicott Settlement"
+ },
+ {
+ "name": "HIP 43310",
+ "pos_x": 115.71875,
+ "pos_y": 15.59375,
+ "pos_z": -29.875,
+ "stations": "MacKellar Dock,Oppenheimer Holdings,Song Installation,Borel Silo"
+ },
+ {
+ "name": "HIP 4332",
+ "pos_x": 38.6875,
+ "pos_y": -238.5,
+ "pos_z": 18.65625,
+ "stations": "Poyser Colony,Samos Enterprise,Lagadha Stop"
+ },
+ {
+ "name": "HIP 43358",
+ "pos_x": 136.09375,
+ "pos_y": 11.71875,
+ "pos_z": -26.71875,
+ "stations": "Watts Terminal"
+ },
+ {
+ "name": "HIP 43363",
+ "pos_x": 98.75,
+ "pos_y": 45.75,
+ "pos_z": -64.09375,
+ "stations": "Barr Port,Britnev Landing,Gresh Relay,Obruchev Penal colony"
+ },
+ {
+ "name": "HIP 43477",
+ "pos_x": -50.59375,
+ "pos_y": 80.25,
+ "pos_z": -91.15625,
+ "stations": "Hilbert Dock,Crowley Dock,Varthema Settlement"
+ },
+ {
+ "name": "HIP 43552",
+ "pos_x": 58.375,
+ "pos_y": 92.78125,
+ "pos_z": -114.5625,
+ "stations": "Beatty's Claim,Litke Base"
+ },
+ {
+ "name": "HIP 43634",
+ "pos_x": 17.09375,
+ "pos_y": 133.59375,
+ "pos_z": -158.34375,
+ "stations": "Ford Vision"
+ },
+ {
+ "name": "HIP 43670",
+ "pos_x": 34.65625,
+ "pos_y": 83.5625,
+ "pos_z": -100.375,
+ "stations": "Shaara Gateway,Hoyle Orbital,Emshwiller Station,Holden Dock,Menezes City,Rochon Port,von Bellingshausen Dock,Young Silo,Rosenberg Settlement,Oramus Terminal,Aldiss Ring"
+ },
+ {
+ "name": "HIP 43756",
+ "pos_x": 67.28125,
+ "pos_y": 71.78125,
+ "pos_z": -88.15625,
+ "stations": "Jensen Ring,Hope Port,Fourneyron Enterprise"
+ },
+ {
+ "name": "HIP 43760",
+ "pos_x": 147.65625,
+ "pos_y": -31.8125,
+ "pos_z": 26.75,
+ "stations": "Jones Relay,Gregory Works"
+ },
+ {
+ "name": "HIP 43766",
+ "pos_x": 42.96875,
+ "pos_y": 99,
+ "pos_z": -117.96875,
+ "stations": "Dyomin Silo,Helmholtz Platform,Merbold Landing,Frobenius Terminal"
+ },
+ {
+ "name": "HIP 43841",
+ "pos_x": -44.84375,
+ "pos_y": 85.0625,
+ "pos_z": -95.40625,
+ "stations": "Fermat Stop"
+ },
+ {
+ "name": "HIP 43845",
+ "pos_x": 170.21875,
+ "pos_y": -64.90625,
+ "pos_z": 63.96875,
+ "stations": "Karman Dock,Tomita Terminal,Fedden Installation,Barcelo Bastion"
+ },
+ {
+ "name": "HIP 43880",
+ "pos_x": 130.46875,
+ "pos_y": 12.71875,
+ "pos_z": -22.75,
+ "stations": "Young Port,Asprin Penal colony"
+ },
+ {
+ "name": "HIP 43886",
+ "pos_x": -91.53125,
+ "pos_y": 69.34375,
+ "pos_z": -74.1875,
+ "stations": "Tudela Refinery"
+ },
+ {
+ "name": "HIP 4392",
+ "pos_x": 53.96875,
+ "pos_y": -222.625,
+ "pos_z": 28.625,
+ "stations": "Orban Vision,Sarrantonio Relay,Giger Colony,Biermann Station"
+ },
+ {
+ "name": "HIP 43947",
+ "pos_x": 116.25,
+ "pos_y": 21.96875,
+ "pos_z": -32,
+ "stations": "Slade Terminal,Person Settlement"
+ },
+ {
+ "name": "HIP 43973",
+ "pos_x": 149.0625,
+ "pos_y": 74.40625,
+ "pos_z": -93.75,
+ "stations": "Marley Mines"
+ },
+ {
+ "name": "HIP 44030",
+ "pos_x": 126.84375,
+ "pos_y": 75.5,
+ "pos_z": -92.90625,
+ "stations": "Schachner Installation,Murphy Beacon,Benford Depot,Viete Point"
+ },
+ {
+ "name": "HIP 44059",
+ "pos_x": 126.8125,
+ "pos_y": 52.8125,
+ "pos_z": -66.65625,
+ "stations": "Tennyson d'Eyncourt Orbital,Soto Station"
+ },
+ {
+ "name": "HIP 44089",
+ "pos_x": 35.53125,
+ "pos_y": 78.90625,
+ "pos_z": -91.15625,
+ "stations": "Collins Survey"
+ },
+ {
+ "name": "HIP 44115",
+ "pos_x": 11.375,
+ "pos_y": 79.65625,
+ "pos_z": -90.625,
+ "stations": "Bakewell Station,Kinsey Beacon"
+ },
+ {
+ "name": "HIP 44137",
+ "pos_x": 96.03125,
+ "pos_y": 90.6875,
+ "pos_z": -106.96875,
+ "stations": "Nolan Colony,Elcano Colony,Alexandrov Observatory"
+ },
+ {
+ "name": "HIP 44169",
+ "pos_x": 23.15625,
+ "pos_y": 124.53125,
+ "pos_z": -141.25,
+ "stations": "McDonald Terminal,Taylor Platform,Wilder Installation"
+ },
+ {
+ "name": "HIP 44176",
+ "pos_x": 27.59375,
+ "pos_y": 88.71875,
+ "pos_z": -101.0625,
+ "stations": "Noether Vision,Puleston Station,Le Guin Works,Carlisle Station,Nordenskiold Vision"
+ },
+ {
+ "name": "HIP 44212",
+ "pos_x": 117.25,
+ "pos_y": 60.75,
+ "pos_z": -73.21875,
+ "stations": "H. G. Wells' Folly,Blackman Terminal,Boole Vision,Navigator Landing"
+ },
+ {
+ "name": "HIP 44387",
+ "pos_x": 34.09375,
+ "pos_y": 79.40625,
+ "pos_z": -88.9375,
+ "stations": "Priestley Orbital,McArthur Platform,Thagard Port,Conklin Keep,Pellegrino Prospect"
+ },
+ {
+ "name": "HIP 44463",
+ "pos_x": -94.28125,
+ "pos_y": 93.625,
+ "pos_z": -100.0625,
+ "stations": "Lessing Relay"
+ },
+ {
+ "name": "HIP 44520",
+ "pos_x": 115,
+ "pos_y": 94.9375,
+ "pos_z": -106.8125,
+ "stations": "Bradshaw Base,Eisenstein Landing"
+ },
+ {
+ "name": "HIP 44537",
+ "pos_x": 3.53125,
+ "pos_y": 107.78125,
+ "pos_z": -117.75,
+ "stations": "vo Relay,Tull Horizons"
+ },
+ {
+ "name": "HIP 44560",
+ "pos_x": 136.40625,
+ "pos_y": 47.6875,
+ "pos_z": -55.21875,
+ "stations": "Lawhead Landing,Sanger Asylum"
+ },
+ {
+ "name": "HIP 44586",
+ "pos_x": 47.75,
+ "pos_y": 93.15625,
+ "pos_z": -102.4375,
+ "stations": "Fremont Platform,Biggle Survey"
+ },
+ {
+ "name": "HIP 44610",
+ "pos_x": -82.625,
+ "pos_y": 87.65625,
+ "pos_z": -93.375,
+ "stations": "Drake Orbital,O'Leary Works,Andrews Survey,Phillifent Hub"
+ },
+ {
+ "name": "HIP 44638",
+ "pos_x": -36.875,
+ "pos_y": 103.3125,
+ "pos_z": -111.125,
+ "stations": "Rontgen Landing,Selye Point"
+ },
+ {
+ "name": "HIP 44687",
+ "pos_x": 26.3125,
+ "pos_y": 87.15625,
+ "pos_z": -94.40625,
+ "stations": "Scithers Freeport"
+ },
+ {
+ "name": "HIP 44719",
+ "pos_x": 119.65625,
+ "pos_y": 20.25,
+ "pos_z": -23.625,
+ "stations": "Bering Barracks,Cook Refinery"
+ },
+ {
+ "name": "HIP 44727",
+ "pos_x": -14.3125,
+ "pos_y": 106.375,
+ "pos_z": -114.125,
+ "stations": "Comino Station,Houssay Horizons,Carey Hub"
+ },
+ {
+ "name": "HIP 44777",
+ "pos_x": 101.25,
+ "pos_y": 76.71875,
+ "pos_z": -83.28125,
+ "stations": "Moorcock Terminal,Minkowski Base"
+ },
+ {
+ "name": "HIP 44791",
+ "pos_x": 7.625,
+ "pos_y": 93.15625,
+ "pos_z": -99.65625,
+ "stations": "Wilson Depot,Macan Settlement,Fermi Port,Scheutz Dock,Fettman Enterprise,Haipeng Plant"
+ },
+ {
+ "name": "HIP 44811",
+ "pos_x": 132.84375,
+ "pos_y": -4.40625,
+ "pos_z": 3.34375,
+ "stations": "Mullane Terminal,Metcalf Station,Popov Terminal,Poleshchuk Gateway,Samuda Base,Garan Ring,Doi Ring,Norman Base,Perry Base,Rescue Ship - Metcalf Station"
+ },
+ {
+ "name": "HIP 44860",
+ "pos_x": 101.6875,
+ "pos_y": 44.78125,
+ "pos_z": -48.46875,
+ "stations": "Wilson Terminal,McMullen Depot,Adams Platform"
+ },
+ {
+ "name": "HIP 44890",
+ "pos_x": 104.875,
+ "pos_y": 66.75,
+ "pos_z": -71.53125,
+ "stations": "Laing Plant,Williams Dock,Sarafanov Plant"
+ },
+ {
+ "name": "HIP 44997",
+ "pos_x": 78.53125,
+ "pos_y": 104.15625,
+ "pos_z": -109.90625,
+ "stations": "Ohm Station,Varley Bastion,Lerner Terminal"
+ },
+ {
+ "name": "HIP 45096",
+ "pos_x": 133.40625,
+ "pos_y": -10.9375,
+ "pos_z": 11.90625,
+ "stations": "Babcock Beacon,Dutton Depot,Hopkinson Penal colony"
+ },
+ {
+ "name": "HIP 45183",
+ "pos_x": 174.71875,
+ "pos_y": -79.96875,
+ "pos_z": 84.40625,
+ "stations": "Kojima Prospect,van der Riet Woolley Oasis,Fox Survey"
+ },
+ {
+ "name": "HIP 4525",
+ "pos_x": -18.09375,
+ "pos_y": -230.09375,
+ "pos_z": -21.875,
+ "stations": "Townes Works,Saha Base"
+ },
+ {
+ "name": "HIP 45251",
+ "pos_x": 116.0625,
+ "pos_y": 25.46875,
+ "pos_z": -24.75,
+ "stations": "Parker Enterprise,Obruchev Silo,Gemar Hub"
+ },
+ {
+ "name": "HIP 45274",
+ "pos_x": -15.28125,
+ "pos_y": 118.65625,
+ "pos_z": -122.21875,
+ "stations": "Reed Depot,Clairaut Works,Kagan Dock"
+ },
+ {
+ "name": "HIP 4536",
+ "pos_x": 104.34375,
+ "pos_y": -207.15625,
+ "pos_z": 61.6875,
+ "stations": "Alexander Settlement"
+ },
+ {
+ "name": "HIP 45389",
+ "pos_x": 9.4375,
+ "pos_y": 116.25,
+ "pos_z": -118.34375,
+ "stations": "Burkin Platform,Macomb Port,Cook Settlement"
+ },
+ {
+ "name": "HIP 45401",
+ "pos_x": -6.53125,
+ "pos_y": 120,
+ "pos_z": -122.34375,
+ "stations": "Viete Oasis,Regiomontanus Colony"
+ },
+ {
+ "name": "HIP 45406",
+ "pos_x": 47.6875,
+ "pos_y": 100.40625,
+ "pos_z": -101.28125,
+ "stations": "Harris Dock,Boswell Camp"
+ },
+ {
+ "name": "HIP 45734",
+ "pos_x": 161.21875,
+ "pos_y": -61.84375,
+ "pos_z": 67.21875,
+ "stations": "Lundmark Point"
+ },
+ {
+ "name": "HIP 45822",
+ "pos_x": 16.9375,
+ "pos_y": 85.5,
+ "pos_z": -83.34375,
+ "stations": "Steiner Dock"
+ },
+ {
+ "name": "HIP 45870",
+ "pos_x": 19.6875,
+ "pos_y": 116.875,
+ "pos_z": -113.5625,
+ "stations": "Mouhot Enterprise,Weber Settlement,Hassanein Relay"
+ },
+ {
+ "name": "HIP 45957",
+ "pos_x": 118.09375,
+ "pos_y": 27.1875,
+ "pos_z": -20.6875,
+ "stations": "Veach City,Buckey Terminal,Pauling Station,Fleming Station,Shalatula Installation,Coulter Point,Hawking Station,Auer Relay,Delany Survey,Laval Beacon"
+ },
+ {
+ "name": "HIP 46007",
+ "pos_x": 137,
+ "pos_y": 41.3125,
+ "pos_z": -32.96875,
+ "stations": "Bass Dock,Corte-Real Ring,Anderson Hub,Fontenay Vision,Tilman Prospect"
+ },
+ {
+ "name": "HIP 46023",
+ "pos_x": 21.40625,
+ "pos_y": 98.9375,
+ "pos_z": -94.53125,
+ "stations": "Sadi Carnot Orbital"
+ },
+ {
+ "name": "HIP 46034",
+ "pos_x": 110.09375,
+ "pos_y": 76.90625,
+ "pos_z": -68.4375,
+ "stations": "Egan Hangar,Weiss Dock,Forbes Oasis"
+ },
+ {
+ "name": "HIP 46046",
+ "pos_x": 143.96875,
+ "pos_y": -55.375,
+ "pos_z": 61.0625,
+ "stations": "Ron Hubbard Settlement,Evans Terminal,Westphal Port,Alcock Hub,Serling Landing"
+ },
+ {
+ "name": "HIP 46110",
+ "pos_x": 4.9375,
+ "pos_y": 117.3125,
+ "pos_z": -112.34375,
+ "stations": "Le Guin Terminal,King Hub,Shatner Orbital,Alvares' Folly"
+ },
+ {
+ "name": "HIP 4617",
+ "pos_x": -123.6875,
+ "pos_y": 55.34375,
+ "pos_z": -81.34375,
+ "stations": "Gunn Relay"
+ },
+ {
+ "name": "HIP 46179",
+ "pos_x": 167.3125,
+ "pos_y": 2.71875,
+ "pos_z": 7.28125,
+ "stations": "Slusser Co-operative,Rocklynne Relay"
+ },
+ {
+ "name": "HIP 46236",
+ "pos_x": 143.90625,
+ "pos_y": -4.84375,
+ "pos_z": 13.4375,
+ "stations": "Yamazaki Station,Bokeili Palace,Lonchakov Ring"
+ },
+ {
+ "name": "HIP 46298",
+ "pos_x": -97.75,
+ "pos_y": 92.4375,
+ "pos_z": -93.78125,
+ "stations": "Lenthall Escape,Staden Laboratory,Ross Enterprise"
+ },
+ {
+ "name": "HIP 4637",
+ "pos_x": -143,
+ "pos_y": -42.125,
+ "pos_z": -99.3125,
+ "stations": "Popper Port,Delany Orbital,Lem Barracks"
+ },
+ {
+ "name": "HIP 46388",
+ "pos_x": 160.6875,
+ "pos_y": -14.9375,
+ "pos_z": 25.0625,
+ "stations": "Goodman Landing,Littrow Terminal,Mikulin Platform"
+ },
+ {
+ "name": "HIP 46407",
+ "pos_x": 169.90625,
+ "pos_y": -48.25,
+ "pos_z": 57.09375,
+ "stations": "Wirtanen Landing,Alexandria's Inheritance,Vess Platform"
+ },
+ {
+ "name": "HIP 46422",
+ "pos_x": 120.875,
+ "pos_y": -24.28125,
+ "pos_z": 31.25,
+ "stations": "Gustafsson Orbital,Merrill Ring,Sauma Hub,Wolfe Prospect"
+ },
+ {
+ "name": "HIP 46478",
+ "pos_x": 43.34375,
+ "pos_y": 83.96875,
+ "pos_z": -75.09375,
+ "stations": "Jemison Station,Gagarin Vision"
+ },
+ {
+ "name": "HIP 46523",
+ "pos_x": 137.0625,
+ "pos_y": 11.625,
+ "pos_z": -0.46875,
+ "stations": "Gurragchaa City,Ohm Dock,Bixby Survey,Clark Dock,Midgley Hub,Kovalevsky Orbital,Shaver Prospect,Bennett Station,Gubarev Camp,Card Beacon"
+ },
+ {
+ "name": "HIP 46598",
+ "pos_x": 97.28125,
+ "pos_y": 81.3125,
+ "pos_z": -67.4375,
+ "stations": "Richards Dock,Boe Port"
+ },
+ {
+ "name": "HIP 4663",
+ "pos_x": 20.3125,
+ "pos_y": -255.84375,
+ "pos_z": 0.71875,
+ "stations": "Carsono Survey,Flamsteed Platform"
+ },
+ {
+ "name": "HIP 46662",
+ "pos_x": 53.125,
+ "pos_y": 92.25,
+ "pos_z": -80.5,
+ "stations": "Dedekind Terminal"
+ },
+ {
+ "name": "HIP 4668",
+ "pos_x": 93.875,
+ "pos_y": -185.28125,
+ "pos_z": 54.4375,
+ "stations": "Curtiss Orbital,Moore Vista,Koishikawa Hub,Lee Dock,McKie Hub,Bouvard Settlement,Weaver Orbital,Sheepshanks Hub,Graham Port,Mil Terminal,Seamans Orbital,Spurzem Palace,Parker Enterprise"
+ },
+ {
+ "name": "HIP 46685",
+ "pos_x": 160.4375,
+ "pos_y": 57.125,
+ "pos_z": -39.28125,
+ "stations": "Franklin Survey"
+ },
+ {
+ "name": "HIP 46903",
+ "pos_x": -11.625,
+ "pos_y": 109.28125,
+ "pos_z": -99.8125,
+ "stations": "Herjulfsson Station,Wollheim Stop"
+ },
+ {
+ "name": "HIP 4699",
+ "pos_x": -24.21875,
+ "pos_y": -135.46875,
+ "pos_z": -23.75,
+ "stations": "Belyayev Survey"
+ },
+ {
+ "name": "HIP 47002",
+ "pos_x": 135.96875,
+ "pos_y": 51.875,
+ "pos_z": -33.46875,
+ "stations": "Fiennes Landing,Bierce's Inheritance,Shunn Holdings"
+ },
+ {
+ "name": "HIP 47050",
+ "pos_x": 149.5,
+ "pos_y": 94.5625,
+ "pos_z": -69.8125,
+ "stations": "Tarentum Mine"
+ },
+ {
+ "name": "HIP 47058",
+ "pos_x": 93.75,
+ "pos_y": 95.03125,
+ "pos_z": -75.59375,
+ "stations": "Ryazanski Enterprise,Armstrong Outpost"
+ },
+ {
+ "name": "HIP 47110",
+ "pos_x": 9.0625,
+ "pos_y": 94.46875,
+ "pos_z": -83,
+ "stations": "Francisco de Almeida Ring,Shiner Penal colony,Lavrador Laboratory"
+ },
+ {
+ "name": "HIP 47156",
+ "pos_x": 132.75,
+ "pos_y": 55.09375,
+ "pos_z": -35.21875,
+ "stations": "Thuot Hangar,Hoften Base"
+ },
+ {
+ "name": "HIP 47255",
+ "pos_x": 142.6875,
+ "pos_y": 29.28125,
+ "pos_z": -10.40625,
+ "stations": "Lewitt Dock,Williams City,Toll Station,Constantine Dock,Rusch Hub"
+ },
+ {
+ "name": "HIP 47263",
+ "pos_x": 169.4375,
+ "pos_y": -16,
+ "pos_z": 32.1875,
+ "stations": "Navigator Penal colony,Nicholson Observatory"
+ },
+ {
+ "name": "HIP 4727",
+ "pos_x": -91.96875,
+ "pos_y": -126.71875,
+ "pos_z": -69.8125,
+ "stations": "Fairbairn Colony,Nemere Orbital,Obruchev Depot"
+ },
+ {
+ "name": "HIP 47292",
+ "pos_x": 114.1875,
+ "pos_y": 109.5,
+ "pos_z": -83.5,
+ "stations": "Asimov Settlement,Chasles Settlement,Sawyer Terminal"
+ },
+ {
+ "name": "HIP 47328",
+ "pos_x": 131.5,
+ "pos_y": 22.40625,
+ "pos_z": -5.15625,
+ "stations": "Werner von Siemens Gateway,Warner Depot,Baxter Beacon,Cseszneky Lab"
+ },
+ {
+ "name": "HIP 47403",
+ "pos_x": 17.75,
+ "pos_y": 121,
+ "pos_z": -103.09375,
+ "stations": "Sargent Vision"
+ },
+ {
+ "name": "HIP 47436",
+ "pos_x": 17.4375,
+ "pos_y": 121,
+ "pos_z": -102.78125,
+ "stations": "Cameron Terminal,Walz Colony,Volta Enterprise"
+ },
+ {
+ "name": "HIP 47440",
+ "pos_x": 120.875,
+ "pos_y": 71.96875,
+ "pos_z": -48.4375,
+ "stations": "Serling Vision,Weyl Arsenal"
+ },
+ {
+ "name": "HIP 47468",
+ "pos_x": 62.9375,
+ "pos_y": 91.53125,
+ "pos_z": -71.59375,
+ "stations": "Boucher Market,Klein Station,Smith Station,Lehtonen Hub,Hui City,Matheson Port,Barnwell Dock"
+ },
+ {
+ "name": "HIP 4747",
+ "pos_x": -36.78125,
+ "pos_y": -114.625,
+ "pos_z": -31.65625,
+ "stations": "Royo Terminal,Vizcaino Base"
+ },
+ {
+ "name": "HIP 47490",
+ "pos_x": 132.34375,
+ "pos_y": -18.40625,
+ "pos_z": 31.34375,
+ "stations": "West Colony"
+ },
+ {
+ "name": "HIP 4764",
+ "pos_x": 52.75,
+ "pos_y": -180.09375,
+ "pos_z": 25.78125,
+ "stations": "Kopal Vision,Michalitsianos Prospect,Cochrane Refinery,Silves Horizons,Bliss Port"
+ },
+ {
+ "name": "HIP 47808",
+ "pos_x": 126.34375,
+ "pos_y": 28.65625,
+ "pos_z": -7.46875,
+ "stations": "Virts Hub,Skripochka Port,Janszoon Relay,Lavoisier Colony"
+ },
+ {
+ "name": "HIP 47839",
+ "pos_x": 167.75,
+ "pos_y": 8.5625,
+ "pos_z": 14.96875,
+ "stations": "Sagan Orbital,Hamilton Oasis,Dalmas Beacon,Stasheff's Progress"
+ },
+ {
+ "name": "HIP 47919",
+ "pos_x": 166.25,
+ "pos_y": 39.8125,
+ "pos_z": -10.59375,
+ "stations": "Vinge Camp,Maine Base,Bass' Claim"
+ },
+ {
+ "name": "HIP 4794",
+ "pos_x": 44.90625,
+ "pos_y": -243.1875,
+ "pos_z": 16.4375,
+ "stations": "Hickman Orbital,Mayer Port,Smirnova Vision,McNaught Laboratory,Soper Barracks"
+ },
+ {
+ "name": "HIP 47947",
+ "pos_x": 163.1875,
+ "pos_y": 28.28125,
+ "pos_z": -1.28125,
+ "stations": "Chamitoff Port,Nikolayev Colony"
+ },
+ {
+ "name": "HIP 47957",
+ "pos_x": 156.28125,
+ "pos_y": 57.40625,
+ "pos_z": -26.28125,
+ "stations": "Bogdanov Station,Vancouver Port,Ford Installation"
+ },
+ {
+ "name": "HIP 48025",
+ "pos_x": 97.65625,
+ "pos_y": 99.6875,
+ "pos_z": -68.5,
+ "stations": "Kendrick Hangar,Eddington Orbital"
+ },
+ {
+ "name": "HIP 48047",
+ "pos_x": 172.1875,
+ "pos_y": 60.9375,
+ "pos_z": -25.8125,
+ "stations": "Cavendish Orbital,Zajdel Landing,Plante Hub,Fearn Base"
+ },
+ {
+ "name": "HIP 48072",
+ "pos_x": 120.875,
+ "pos_y": 25.25,
+ "pos_z": -3.53125,
+ "stations": "Doctorow Ring"
+ },
+ {
+ "name": "HIP 48095",
+ "pos_x": 133.40625,
+ "pos_y": -44.15625,
+ "pos_z": 55.25,
+ "stations": "Blackwell Dock,Jernigan Station,Carr Plant,Musgrave Port"
+ },
+ {
+ "name": "HIP 48125",
+ "pos_x": 120.9375,
+ "pos_y": 46.84375,
+ "pos_z": -20.78125,
+ "stations": "Taylor Refinery"
+ },
+ {
+ "name": "HIP 48141",
+ "pos_x": 132.84375,
+ "pos_y": 29.96875,
+ "pos_z": -5.21875,
+ "stations": "Yourkevitch City,Popov Installation,Andree City,Henson Installation,Fernandez Prospect"
+ },
+ {
+ "name": "HIP 48165",
+ "pos_x": 77.25,
+ "pos_y": 96.09375,
+ "pos_z": -67.03125,
+ "stations": "Apgar Platform,Bayliss Station,Vonnegut Silo"
+ },
+ {
+ "name": "HIP 48248",
+ "pos_x": 50.90625,
+ "pos_y": 135.03125,
+ "pos_z": -101.59375,
+ "stations": "Crown Hub,Tavernier Dock,Beatty Point,Finch Vision"
+ },
+ {
+ "name": "HIP 48365",
+ "pos_x": 113.65625,
+ "pos_y": 100.1875,
+ "pos_z": -62.6875,
+ "stations": "Barcelos Platform,Budrys' Exile,Kononenko Installation"
+ },
+ {
+ "name": "HIP 48391",
+ "pos_x": -21.03125,
+ "pos_y": 112.90625,
+ "pos_z": -93.53125,
+ "stations": "Bushkov City,Ore Orbital,Wollheim Station,Platt Enterprise"
+ },
+ {
+ "name": "HIP 48504",
+ "pos_x": 77.9375,
+ "pos_y": 141.84375,
+ "pos_z": -99.625,
+ "stations": "Wnuk-Lipinski Port,Andersson Relay"
+ },
+ {
+ "name": "HIP 48524",
+ "pos_x": 67.3125,
+ "pos_y": 111.59375,
+ "pos_z": -77.1875,
+ "stations": "Schmitz Prospect,Hogan Freeport,Fife Bastion"
+ },
+ {
+ "name": "HIP 48592",
+ "pos_x": 128,
+ "pos_y": 83.1875,
+ "pos_z": -44.28125,
+ "stations": "Salak Station,Kingsbury Platform"
+ },
+ {
+ "name": "HIP 4864",
+ "pos_x": 100.40625,
+ "pos_y": -199.28125,
+ "pos_z": 56.46875,
+ "stations": "Deutsch Terminal,Valigursky's Folly"
+ },
+ {
+ "name": "HIP 48680",
+ "pos_x": 107.1875,
+ "pos_y": 59.125,
+ "pos_z": -28.0625,
+ "stations": "Clifton Platform,Shapiro Platform"
+ },
+ {
+ "name": "HIP 48691",
+ "pos_x": -86.34375,
+ "pos_y": 118.3125,
+ "pos_z": -106.65625,
+ "stations": "Gaiman Dock,Phillips Vision,Howard Vision,Young Hub"
+ },
+ {
+ "name": "HIP 48701",
+ "pos_x": 8.21875,
+ "pos_y": 116.875,
+ "pos_z": -89.53125,
+ "stations": "Bushkov Station"
+ },
+ {
+ "name": "HIP 48754",
+ "pos_x": 93.40625,
+ "pos_y": 70,
+ "pos_z": -38.21875,
+ "stations": "Gaspar de Lemos Point,Wait Colburn Dock,Brooks Gateway,Haarsma Survey"
+ },
+ {
+ "name": "HIP 48768",
+ "pos_x": 91.53125,
+ "pos_y": 104.4375,
+ "pos_z": -65.03125,
+ "stations": "Aikin Relay"
+ },
+ {
+ "name": "HIP 4877",
+ "pos_x": 99,
+ "pos_y": -168.09375,
+ "pos_z": 57.375,
+ "stations": "Sternbach Station,Hughes Vista"
+ },
+ {
+ "name": "HIP 4878",
+ "pos_x": -86.6875,
+ "pos_y": -149.0625,
+ "pos_z": -69.375,
+ "stations": "Saberhagen Base"
+ },
+ {
+ "name": "HIP 48786",
+ "pos_x": 49.71875,
+ "pos_y": 104.84375,
+ "pos_z": -72.375,
+ "stations": "Beaumont Vision,Smith Installation"
+ },
+ {
+ "name": "HIP 48854",
+ "pos_x": -98.90625,
+ "pos_y": 103.28125,
+ "pos_z": -96.59375,
+ "stations": "Norman Survey,Walters Vista"
+ },
+ {
+ "name": "HIP 48887",
+ "pos_x": -29.5625,
+ "pos_y": 127.53125,
+ "pos_z": -102.84375,
+ "stations": "Marusek Co-operative"
+ },
+ {
+ "name": "HIP 4892",
+ "pos_x": 33.84375,
+ "pos_y": -194.71875,
+ "pos_z": 10.65625,
+ "stations": "Flammarion Terminal,Sato Barracks,Oxley Terminal,Laurent Vision"
+ },
+ {
+ "name": "HIP 48961",
+ "pos_x": 34.03125,
+ "pos_y": 115.25,
+ "pos_z": -81.53125,
+ "stations": "Sargent Penal colony"
+ },
+ {
+ "name": "HIP 4907",
+ "pos_x": -68.3125,
+ "pos_y": 9.625,
+ "pos_z": -46.5,
+ "stations": "Macgregor City,Schachner Horizons,Leslie Hub"
+ },
+ {
+ "name": "HIP 49070",
+ "pos_x": -34.03125,
+ "pos_y": 126.84375,
+ "pos_z": -101.65625,
+ "stations": "Gottlob Frege Ring,Hui Orbital,Carrasco Mines,Roberts Installation,Caidin Installation"
+ },
+ {
+ "name": "HIP 49080",
+ "pos_x": 86.6875,
+ "pos_y": 118.90625,
+ "pos_z": -73.25,
+ "stations": "Wiener Gateway,Mitchell Enterprise,Robson Ring,Serre Point"
+ },
+ {
+ "name": "HIP 4909",
+ "pos_x": -40.40625,
+ "pos_y": -175.21875,
+ "pos_z": -39.40625,
+ "stations": "Yize Vision"
+ },
+ {
+ "name": "HIP 49102",
+ "pos_x": -14.28125,
+ "pos_y": 131.09375,
+ "pos_z": -100.9375,
+ "stations": "Carr Relay"
+ },
+ {
+ "name": "HIP 49104",
+ "pos_x": 96.5625,
+ "pos_y": 118.90625,
+ "pos_z": -71.15625,
+ "stations": "Huberath Dock,Moore Landing,Kingsmill Bastion"
+ },
+ {
+ "name": "HIP 49161",
+ "pos_x": 115.09375,
+ "pos_y": 84.46875,
+ "pos_z": -41.34375,
+ "stations": "Shiras Terminal,Hedin Point"
+ },
+ {
+ "name": "HIP 49248",
+ "pos_x": 131.71875,
+ "pos_y": 12.5625,
+ "pos_z": 16,
+ "stations": "Feynman Terminal,Liouville Installation,Creamer Port"
+ },
+ {
+ "name": "HIP 49285",
+ "pos_x": 143.4375,
+ "pos_y": 55.4375,
+ "pos_z": -13.15625,
+ "stations": "Taine Hub,Gold City,Ings Station,Slade Survey"
+ },
+ {
+ "name": "HIP 493",
+ "pos_x": -83.5625,
+ "pos_y": -82.90625,
+ "pos_z": -28.625,
+ "stations": "Siodmak City,Okorafor Station,Sellings Ring,Whitelaw Gateway"
+ },
+ {
+ "name": "HIP 49308",
+ "pos_x": 7.21875,
+ "pos_y": 110.53125,
+ "pos_z": -80,
+ "stations": "Crook Mines,Malchiodi Terminal,Chang-Diaz Point"
+ },
+ {
+ "name": "HIP 4934",
+ "pos_x": 123.21875,
+ "pos_y": -220.25,
+ "pos_z": 70.28125,
+ "stations": "Babcock Legacy"
+ },
+ {
+ "name": "HIP 494",
+ "pos_x": 132.09375,
+ "pos_y": -188.9375,
+ "pos_z": 106.21875,
+ "stations": "Swift Stop,Meech Terminal,Panshin Penal colony"
+ },
+ {
+ "name": "HIP 495",
+ "pos_x": -83.40625,
+ "pos_y": -83.1875,
+ "pos_z": -28.5,
+ "stations": "Townshend Orbital,Zetford Silo,Potrykus Platform"
+ },
+ {
+ "name": "HIP 49580",
+ "pos_x": 25.53125,
+ "pos_y": 103.5,
+ "pos_z": -69.21875,
+ "stations": "Padalka Enterprise,Sullivan Ring,Cameron Orbital,Rand Enterprise"
+ },
+ {
+ "name": "HIP 49599",
+ "pos_x": 135.4375,
+ "pos_y": 35.90625,
+ "pos_z": 2.0625,
+ "stations": "Moore Orbital,Nelson Dock,Tarelkin Terminal,Ride Ring,Andreas Dock,Crampton Enterprise,Thorne Ring"
+ },
+ {
+ "name": "HIP 49615",
+ "pos_x": 111.4375,
+ "pos_y": 95.25,
+ "pos_z": -45.40625,
+ "stations": "Poleshchuk Terminal,McCaffrey Relay,Vittori Settlement,Eilenberg Arsenal"
+ },
+ {
+ "name": "HIP 4964",
+ "pos_x": 50.9375,
+ "pos_y": -166.90625,
+ "pos_z": 23.71875,
+ "stations": "Millosevich Base,Camm Camp"
+ },
+ {
+ "name": "HIP 49668",
+ "pos_x": 105.34375,
+ "pos_y": 59.15625,
+ "pos_z": -20.28125,
+ "stations": "Back Settlement,Tani Terminal,Kondakova City,Smith Enterprise,Eisenstein Settlement"
+ },
+ {
+ "name": "HIP 49674",
+ "pos_x": 97.84375,
+ "pos_y": 72.625,
+ "pos_z": -31.375,
+ "stations": "Deere Station,Ramanujan Port,Makeev's Claim"
+ },
+ {
+ "name": "HIP 49694",
+ "pos_x": 109.78125,
+ "pos_y": 119.8125,
+ "pos_z": -62.3125,
+ "stations": "Cowper Terminal,Maury Vision,Sagan Installation"
+ },
+ {
+ "name": "HIP 4974",
+ "pos_x": 17.5625,
+ "pos_y": -234.46875,
+ "pos_z": -4.125,
+ "stations": "Calatrava Oasis,South Station,Flagg Works,Moffitt Beacon"
+ },
+ {
+ "name": "HIP 49767",
+ "pos_x": 133.5625,
+ "pos_y": 45.5625,
+ "pos_z": -3.9375,
+ "stations": "Swanwick Hub,Neumann Dock,Payne Dock,Aitken Station,Gaiman Station,Reis Station,Almagro Orbital"
+ },
+ {
+ "name": "HIP 49771",
+ "pos_x": 85.71875,
+ "pos_y": 157.03125,
+ "pos_z": -92.875,
+ "stations": "Vance's Progress,McDevitt Retreat"
+ },
+ {
+ "name": "HIP 49806",
+ "pos_x": 111.0625,
+ "pos_y": 50.5,
+ "pos_z": -11.90625,
+ "stations": "Pike Orbital,Martinez Vision"
+ },
+ {
+ "name": "HIP 49846",
+ "pos_x": 57.40625,
+ "pos_y": 108.90625,
+ "pos_z": -64.0625,
+ "stations": "Wohler Ring,Fozard Enterprise"
+ },
+ {
+ "name": "HIP 50",
+ "pos_x": 58.15625,
+ "pos_y": -172.34375,
+ "pos_z": 66.875,
+ "stations": "Bauschinger Vision,Whelan Colony,Tyson Dock,Shepard Point"
+ },
+ {
+ "name": "HIP 50013",
+ "pos_x": 114.78125,
+ "pos_y": 67.375,
+ "pos_z": -21.1875,
+ "stations": "Almagro Settlement,Nylund Platform,Crook Arsenal"
+ },
+ {
+ "name": "HIP 50032",
+ "pos_x": 128.1875,
+ "pos_y": 53.8125,
+ "pos_z": -8.6875,
+ "stations": "Barry Terminal,Popov Terminal,Swigert City,Shosuke Point"
+ },
+ {
+ "name": "HIP 5004",
+ "pos_x": 28.6875,
+ "pos_y": -208.5625,
+ "pos_z": 5.03125,
+ "stations": "Bahcall Dock"
+ },
+ {
+ "name": "HIP 50054",
+ "pos_x": 76.15625,
+ "pos_y": 113.25,
+ "pos_z": -61.09375,
+ "stations": "Thorne Dock,Veach Horizons"
+ },
+ {
+ "name": "HIP 50084",
+ "pos_x": 122.75,
+ "pos_y": 112.65625,
+ "pos_z": -49.9375,
+ "stations": "Lindsey Platform,McCormick Colony"
+ },
+ {
+ "name": "HIP 50155",
+ "pos_x": 73.96875,
+ "pos_y": 137,
+ "pos_z": -76.90625,
+ "stations": "Mayer Platform"
+ },
+ {
+ "name": "HIP 50176",
+ "pos_x": 159.78125,
+ "pos_y": 71.78125,
+ "pos_z": -12.65625,
+ "stations": "Lawhead Laboratory"
+ },
+ {
+ "name": "HIP 50194",
+ "pos_x": 4.8125,
+ "pos_y": 131.65625,
+ "pos_z": -88.625,
+ "stations": "Anthony Outpost"
+ },
+ {
+ "name": "HIP 50197",
+ "pos_x": 151.21875,
+ "pos_y": -14.0625,
+ "pos_z": 44.09375,
+ "stations": "Heng Gateway,Ibold Prospect"
+ },
+ {
+ "name": "HIP 50216",
+ "pos_x": -9.1875,
+ "pos_y": 118.3125,
+ "pos_z": -82.5625,
+ "stations": "Roosa Platform"
+ },
+ {
+ "name": "HIP 50267",
+ "pos_x": 97.84375,
+ "pos_y": 73.84375,
+ "pos_z": -27.3125,
+ "stations": "Alvarez de Pineda Survey,Bell Orbital,Herrington Station"
+ },
+ {
+ "name": "HIP 50274",
+ "pos_x": 124.46875,
+ "pos_y": -43.8125,
+ "pos_z": 58.4375,
+ "stations": "Grant Terminal"
+ },
+ {
+ "name": "HIP 5031",
+ "pos_x": -40.1875,
+ "pos_y": -114.4375,
+ "pos_z": -36.25,
+ "stations": "Sheepshanks Platform,Harvia Landing,Duque Camp,Nearchus Arsenal"
+ },
+ {
+ "name": "HIP 50352",
+ "pos_x": 133.96875,
+ "pos_y": 111.09375,
+ "pos_z": -43.09375,
+ "stations": "Carrasco Port,Williams Installation,Vonarburg Dock,Narita Dock,Matheson Vision"
+ },
+ {
+ "name": "HIP 50489",
+ "pos_x": 150.5,
+ "pos_y": 47.65625,
+ "pos_z": 4.46875,
+ "stations": "Laliberte Orbital,Pudwill Gorie Orbital,Elion Port,Luiken Beacon,Humphreys Hub"
+ },
+ {
+ "name": "HIP 50506",
+ "pos_x": 22,
+ "pos_y": 132.46875,
+ "pos_z": -82.28125,
+ "stations": "Parise Stop,Culbertson Prison"
+ },
+ {
+ "name": "HIP 50515",
+ "pos_x": 120.4375,
+ "pos_y": 94.125,
+ "pos_z": -33.1875,
+ "stations": "Anderson Installation,Nearchus Horizons"
+ },
+ {
+ "name": "HIP 50534",
+ "pos_x": 99.96875,
+ "pos_y": 77.75,
+ "pos_z": -27.125,
+ "stations": "Linenger Ring,Sullivan Terminal,Franklin Settlement,Acton Observatory"
+ },
+ {
+ "name": "HIP 50660",
+ "pos_x": 21.21875,
+ "pos_y": 129.71875,
+ "pos_z": -79.40625,
+ "stations": "Armstrong Hub"
+ },
+ {
+ "name": "HIP 50661",
+ "pos_x": 172.4375,
+ "pos_y": 106.09375,
+ "pos_z": -26.75,
+ "stations": "Nelson Port,Sternberg Depot"
+ },
+ {
+ "name": "HIP 50694",
+ "pos_x": 126.78125,
+ "pos_y": 8.625,
+ "pos_z": 25.75,
+ "stations": "Zebrowski Enterprise,Mitchell Terminal,Turtledove Arsenal"
+ },
+ {
+ "name": "HIP 50742",
+ "pos_x": -20.5,
+ "pos_y": 129.96875,
+ "pos_z": -89.3125,
+ "stations": "Vinge Horizons,Giles Colony"
+ },
+ {
+ "name": "HIP 50765",
+ "pos_x": 148.21875,
+ "pos_y": -13.6875,
+ "pos_z": 45.875,
+ "stations": "Kapp Hub"
+ },
+ {
+ "name": "HIP 50805",
+ "pos_x": 129.125,
+ "pos_y": 55.125,
+ "pos_z": -3.125,
+ "stations": "Sverdrup City,Ron Hubbard Ring,Morgan Enterprise,Haarsma Terminal,Sverdrup Hub"
+ },
+ {
+ "name": "HIP 50807",
+ "pos_x": 130.96875,
+ "pos_y": 57.5,
+ "pos_z": -4.1875,
+ "stations": "Wright Survey"
+ },
+ {
+ "name": "HIP 50826",
+ "pos_x": 77.90625,
+ "pos_y": 162.96875,
+ "pos_z": -85.1875,
+ "stations": "Farrukh Reach,Busch Vision,Grothendieck Retreat"
+ },
+ {
+ "name": "HIP 50866",
+ "pos_x": 166.875,
+ "pos_y": 22.34375,
+ "pos_z": 27.96875,
+ "stations": "Everest Port,Jones Port"
+ },
+ {
+ "name": "HIP 509",
+ "pos_x": -5.59375,
+ "pos_y": -254.59375,
+ "pos_z": 45.34375,
+ "stations": "Asclepi Relay,Corben Legacy"
+ },
+ {
+ "name": "HIP 5099",
+ "pos_x": 38.875,
+ "pos_y": -174.3125,
+ "pos_z": 13.625,
+ "stations": "Bell Gateway,Parkinson Station,Heiles Station,Foster Penal colony"
+ },
+ {
+ "name": "HIP 50997",
+ "pos_x": 28.34375,
+ "pos_y": 143,
+ "pos_z": -83.03125,
+ "stations": "Maine Port,Barr Depot,Powell Base"
+ },
+ {
+ "name": "HIP 51178",
+ "pos_x": 52.78125,
+ "pos_y": 129.625,
+ "pos_z": -66.59375,
+ "stations": "Schouten Asylum,Simmons Installation"
+ },
+ {
+ "name": "HIP 51197",
+ "pos_x": -59.6875,
+ "pos_y": 88.125,
+ "pos_z": -70.5625,
+ "stations": "Parry's Claim,Allen Mines,Selye Penal colony"
+ },
+ {
+ "name": "HIP 51208",
+ "pos_x": 71.3125,
+ "pos_y": 129.1875,
+ "pos_z": -61.0625,
+ "stations": "Bester Survey,Julian Mine,Desargues Barracks"
+ },
+ {
+ "name": "HIP 51228",
+ "pos_x": 120.90625,
+ "pos_y": 43.4375,
+ "pos_z": 5.40625,
+ "stations": "Kettle Laboratory,Garriott Horizons,Fremont Penal colony"
+ },
+ {
+ "name": "HIP 51280",
+ "pos_x": 71.28125,
+ "pos_y": 99.59375,
+ "pos_z": -42.15625,
+ "stations": "Yamazaki Beacon,McKay Plant,Chiao City"
+ },
+ {
+ "name": "HIP 5134",
+ "pos_x": -72.0625,
+ "pos_y": -165.25,
+ "pos_z": -63.40625,
+ "stations": "Jiushao Settlement,Cook Terminal,Sagan Depot"
+ },
+ {
+ "name": "HIP 51352",
+ "pos_x": 28.03125,
+ "pos_y": 175,
+ "pos_z": -99.40625,
+ "stations": "Russo Oasis"
+ },
+ {
+ "name": "HIP 51372",
+ "pos_x": 151.90625,
+ "pos_y": 100.3125,
+ "pos_z": -19.90625,
+ "stations": "Eskridge Relay"
+ },
+ {
+ "name": "HIP 51487",
+ "pos_x": 31.875,
+ "pos_y": 166.8125,
+ "pos_z": -91.9375,
+ "stations": "Bokeili Settlement,Lessing Vision"
+ },
+ {
+ "name": "HIP 51510",
+ "pos_x": 156.84375,
+ "pos_y": 11.15625,
+ "pos_z": 36.65625,
+ "stations": "Cortes Point,Dozois' Claim,Friend Orbital"
+ },
+ {
+ "name": "HIP 51568",
+ "pos_x": 112.375,
+ "pos_y": 41.65625,
+ "pos_z": 6.40625,
+ "stations": "Piper Station,Grunsfeld Platform,Neville Landing"
+ },
+ {
+ "name": "HIP 51612",
+ "pos_x": 155.3125,
+ "pos_y": 51.5,
+ "pos_z": 12.90625,
+ "stations": "Leavitt Mine"
+ },
+ {
+ "name": "HIP 51652",
+ "pos_x": 27.1875,
+ "pos_y": 151.65625,
+ "pos_z": -82.34375,
+ "stations": "Cowper Prospect,Roons Haven,Rasmussen Base"
+ },
+ {
+ "name": "HIP 51700",
+ "pos_x": -18.875,
+ "pos_y": 100.40625,
+ "pos_z": -64.65625,
+ "stations": "Miklouho-Maclay Ring,Witt Ring,Cook Station,Carlisle City,Stackpole Observatory,Pike Enterprise"
+ },
+ {
+ "name": "HIP 51748",
+ "pos_x": 138.46875,
+ "pos_y": -48.21875,
+ "pos_z": 67.9375,
+ "stations": "Shen Dock"
+ },
+ {
+ "name": "HIP 5178",
+ "pos_x": 92,
+ "pos_y": -184.125,
+ "pos_z": 49.375,
+ "stations": "Young Terminal,Malvern Enterprise"
+ },
+ {
+ "name": "HIP 5183",
+ "pos_x": 33.25,
+ "pos_y": -230.90625,
+ "pos_z": 4.375,
+ "stations": "Oren Mines,Carsono Hub,Roed Odegaard Survey,Ahern Horizons"
+ },
+ {
+ "name": "HIP 5184",
+ "pos_x": 32.1875,
+ "pos_y": -186.3125,
+ "pos_z": 7.21875,
+ "stations": "Lindblad Dock,Lagadha Station,Belyavsky Station,Dittmar Vision,Brill Orbital"
+ },
+ {
+ "name": "HIP 51874",
+ "pos_x": 45.6875,
+ "pos_y": 107.59375,
+ "pos_z": -49.25,
+ "stations": "Carey City,Carleson Depot,Starzl Arena,Dunyach Survey"
+ },
+ {
+ "name": "HIP 51877",
+ "pos_x": 8.71875,
+ "pos_y": 153.84375,
+ "pos_z": -86.8125,
+ "stations": "Shumil Prospect,Delany Mines"
+ },
+ {
+ "name": "HIP 5191",
+ "pos_x": -23.03125,
+ "pos_y": -150.03125,
+ "pos_z": -28.5,
+ "stations": "Nylund Depot,Flagg Relay,Kimura Dock"
+ },
+ {
+ "name": "HIP 51942",
+ "pos_x": 45.78125,
+ "pos_y": 130.125,
+ "pos_z": -61.71875,
+ "stations": "Merle Settlement,Poincare's Exile"
+ },
+ {
+ "name": "HIP 51980",
+ "pos_x": 153.09375,
+ "pos_y": 70.3125,
+ "pos_z": 4.65625,
+ "stations": "Pratchett City,Timofeyevich Settlement"
+ },
+ {
+ "name": "HIP 52045",
+ "pos_x": 148.25,
+ "pos_y": 85.8125,
+ "pos_z": -5,
+ "stations": "Haisheng Mines,Kraepelin Orbital,Newcomen Settlement,Herodotus' Folly"
+ },
+ {
+ "name": "HIP 52065",
+ "pos_x": 165.71875,
+ "pos_y": -89.40625,
+ "pos_z": 100.1875,
+ "stations": "Lyne Prospect,Hind Port,Perga Silo"
+ },
+ {
+ "name": "HIP 52091",
+ "pos_x": 124.34375,
+ "pos_y": 53.34375,
+ "pos_z": 6.75,
+ "stations": "Walker Terminal,Leopold Port,Swanson Market,Wiener Reach,Smith Terminal,Swigert Orbital,Bao Port,Jernigan Hub,Vaez de Torres Vision,Crown Lab"
+ },
+ {
+ "name": "HIP 52137",
+ "pos_x": -45.28125,
+ "pos_y": 137.90625,
+ "pos_z": -91.65625,
+ "stations": "Fearn Landing,Slusser Beacon"
+ },
+ {
+ "name": "HIP 52145",
+ "pos_x": 143.78125,
+ "pos_y": 77.28125,
+ "pos_z": -0.65625,
+ "stations": "Mendez Hub,Banks Relay,Valdes Works"
+ },
+ {
+ "name": "HIP 52237",
+ "pos_x": 110.9375,
+ "pos_y": 52.46875,
+ "pos_z": 4.1875,
+ "stations": "Massimino Plant,Burnell Dock"
+ },
+ {
+ "name": "HIP 52248",
+ "pos_x": 159.09375,
+ "pos_y": 47,
+ "pos_z": 22,
+ "stations": "Nylund Depot,Goldstein Mine"
+ },
+ {
+ "name": "HIP 52278",
+ "pos_x": -44.78125,
+ "pos_y": 106.875,
+ "pos_z": -73.28125,
+ "stations": "Amis Hub,Maury Horizons"
+ },
+ {
+ "name": "HIP 52285",
+ "pos_x": 98,
+ "pos_y": 134.65625,
+ "pos_z": -45.15625,
+ "stations": "Barry's Centre"
+ },
+ {
+ "name": "HIP 52351",
+ "pos_x": 132.75,
+ "pos_y": -45.96875,
+ "pos_z": 66.21875,
+ "stations": "Boyajian Colony,Dunlop Vision,Holberg Base"
+ },
+ {
+ "name": "HIP 52461",
+ "pos_x": 173.6875,
+ "pos_y": -54.5,
+ "pos_z": 83.90625,
+ "stations": "Goldreich Asylum,Roe Stop,Walter Point"
+ },
+ {
+ "name": "HIP 52469",
+ "pos_x": -13.71875,
+ "pos_y": 103.65625,
+ "pos_z": -60.875,
+ "stations": "Ford Platform"
+ },
+ {
+ "name": "HIP 52498",
+ "pos_x": -13.8125,
+ "pos_y": 104.1875,
+ "pos_z": -61,
+ "stations": "Stabenow Landing,Alvares Dock,Sakers Asylum"
+ },
+ {
+ "name": "HIP 5253",
+ "pos_x": -50.6875,
+ "pos_y": -142.78125,
+ "pos_z": -47.9375,
+ "stations": "Palisa Installation,Pacheco Holdings,Lunney Base,McQuarrie Colony"
+ },
+ {
+ "name": "HIP 52545",
+ "pos_x": 145.4375,
+ "pos_y": 78.5,
+ "pos_z": 3.34375,
+ "stations": "Edwards Colony,Viktorenko Dock,King Terminal"
+ },
+ {
+ "name": "HIP 52552",
+ "pos_x": 142.78125,
+ "pos_y": 1.46875,
+ "pos_z": 44.1875,
+ "stations": "Houtman Settlement,Platt Settlement"
+ },
+ {
+ "name": "HIP 52574",
+ "pos_x": 89.5,
+ "pos_y": 124.0625,
+ "pos_z": -38.59375,
+ "stations": "Schroeder Depot"
+ },
+ {
+ "name": "HIP 52649",
+ "pos_x": 129.09375,
+ "pos_y": 96.65625,
+ "pos_z": -10.5625,
+ "stations": "Dunbar City,al-Din Settlement,Chaudhary Station,Chios Relay"
+ },
+ {
+ "name": "HIP 5273",
+ "pos_x": -128.78125,
+ "pos_y": 20.125,
+ "pos_z": -88.84375,
+ "stations": "Iqbal Outpost"
+ },
+ {
+ "name": "HIP 52780",
+ "pos_x": 111.6875,
+ "pos_y": 91.65625,
+ "pos_z": -12.34375,
+ "stations": "Goddard Gateway,al-Din Dock,Rustah Keep,Macedo Settlement"
+ },
+ {
+ "name": "HIP 52828",
+ "pos_x": 140.96875,
+ "pos_y": 77.6875,
+ "pos_z": 4.9375,
+ "stations": "Thurston Beacon,Kerwin Survey"
+ },
+ {
+ "name": "HIP 52891",
+ "pos_x": 52.40625,
+ "pos_y": 153.5625,
+ "pos_z": -62.90625,
+ "stations": "Wnuk-Lipinski Orbital,Norgay Enterprise,McDonald Station,Tall Enterprise"
+ },
+ {
+ "name": "HIP 52923",
+ "pos_x": 157.65625,
+ "pos_y": 59.09375,
+ "pos_z": 21.0625,
+ "stations": "Abe Station,Cartan Port,Patterson Station,Johnson Holdings"
+ },
+ {
+ "name": "HIP 52933",
+ "pos_x": 110.4375,
+ "pos_y": 113.78125,
+ "pos_z": -22.75,
+ "stations": "Bruce Base,Stevens Installation"
+ },
+ {
+ "name": "HIP 5301",
+ "pos_x": -41.15625,
+ "pos_y": -168.5,
+ "pos_z": -44.03125,
+ "stations": "Coppel Prospect,Arfstrom Hub,Ritchey Landing,Sitter Beacon"
+ },
+ {
+ "name": "HIP 53042",
+ "pos_x": -43.28125,
+ "pos_y": 125.34375,
+ "pos_z": -78.5625,
+ "stations": "Brillant Point"
+ },
+ {
+ "name": "HIP 53051",
+ "pos_x": -18,
+ "pos_y": 120.21875,
+ "pos_z": -67.46875,
+ "stations": "Peary Enterprise,West's Folly,LeConte Point"
+ },
+ {
+ "name": "HIP 53070",
+ "pos_x": 47.59375,
+ "pos_y": 136.75,
+ "pos_z": -53.90625,
+ "stations": "Bokeili Depot"
+ },
+ {
+ "name": "HIP 53169",
+ "pos_x": 83.5625,
+ "pos_y": 100.71875,
+ "pos_z": -22.71875,
+ "stations": "Ron Hubbard Port,Ledyard Vision"
+ },
+ {
+ "name": "HIP 53186",
+ "pos_x": 91.3125,
+ "pos_y": 82.875,
+ "pos_z": -11.03125,
+ "stations": "Bartoe Vision,Schmitt Dock"
+ },
+ {
+ "name": "HIP 53189",
+ "pos_x": 23.875,
+ "pos_y": 120.4375,
+ "pos_z": -52.71875,
+ "stations": "Jemison Relay"
+ },
+ {
+ "name": "HIP 53196",
+ "pos_x": 94.25,
+ "pos_y": 98.34375,
+ "pos_z": -17.78125,
+ "stations": "Krylov City,Charnas Station,Steiner Port,Maddox Enterprise,McDonald Orbital,Andrews Station,Gibbs Hub,Stokes Settlement,Chiao Depot,Thirsk Gateway,Bischoff Enterprise"
+ },
+ {
+ "name": "HIP 53206",
+ "pos_x": 101.96875,
+ "pos_y": 77.46875,
+ "pos_z": -4.5625,
+ "stations": "Hire Dock,Amundsen Prospect,Pascal Station,Roosa Station"
+ },
+ {
+ "name": "HIP 53250",
+ "pos_x": 108.84375,
+ "pos_y": 86.71875,
+ "pos_z": -6.53125,
+ "stations": "Garneau Depot,Stephenson Settlement"
+ },
+ {
+ "name": "HIP 53257",
+ "pos_x": -71.09375,
+ "pos_y": 101.0625,
+ "pos_z": -74.8125,
+ "stations": "Rawn Orbital,Hippalus Station,Sleator Dock,Redi Terminal"
+ },
+ {
+ "name": "HIP 53324",
+ "pos_x": 24.5,
+ "pos_y": 160.40625,
+ "pos_z": -71.34375,
+ "stations": "Miklouho-Maclay Station,Yu Installation,Kingsmill Laboratory"
+ },
+ {
+ "name": "HIP 53336",
+ "pos_x": -24.78125,
+ "pos_y": 149.25,
+ "pos_z": -82.625,
+ "stations": "Gibson Mines,Noon Platform"
+ },
+ {
+ "name": "HIP 53401",
+ "pos_x": 149.03125,
+ "pos_y": -41.90625,
+ "pos_z": 72.03125,
+ "stations": "Lalande Port,Tarter Dock,Ashton Bastion"
+ },
+ {
+ "name": "HIP 53424",
+ "pos_x": 110.09375,
+ "pos_y": 120.96875,
+ "pos_z": -21.40625,
+ "stations": "Harvey Hub,Santarem Survey,Moskowitz Hub"
+ },
+ {
+ "name": "HIP 53437",
+ "pos_x": 158.21875,
+ "pos_y": 64.65625,
+ "pos_z": 23.0625,
+ "stations": "Vonnegut Keep"
+ },
+ {
+ "name": "HIP 53452",
+ "pos_x": 38.09375,
+ "pos_y": 159.59375,
+ "pos_z": -65,
+ "stations": "Zajdel City,Spinrad Terminal"
+ },
+ {
+ "name": "HIP 53509",
+ "pos_x": 107.78125,
+ "pos_y": 82.875,
+ "pos_z": -2.78125,
+ "stations": "Glidden Gateway"
+ },
+ {
+ "name": "HIP 53537",
+ "pos_x": 44.4375,
+ "pos_y": 144.28125,
+ "pos_z": -54.4375,
+ "stations": "Fidalgo Survey,Robinson Plant"
+ },
+ {
+ "name": "HIP 53634",
+ "pos_x": -47.375,
+ "pos_y": 112.21875,
+ "pos_z": -70.5625,
+ "stations": "Malocello Hub,Tucker Dock,Cabral Landing"
+ },
+ {
+ "name": "HIP 53639",
+ "pos_x": 104.21875,
+ "pos_y": 111.78125,
+ "pos_z": -16.8125,
+ "stations": "Rosenberg Platform,van Vogt Freeport"
+ },
+ {
+ "name": "HIP 53660",
+ "pos_x": 157.3125,
+ "pos_y": 44.5625,
+ "pos_z": 34.34375,
+ "stations": "Wells Installation,Blish Prospect"
+ },
+ {
+ "name": "HIP 53685",
+ "pos_x": 117.125,
+ "pos_y": 12.03125,
+ "pos_z": 35.8125,
+ "stations": "Bisson Observatory,Lawhead Point,Baturin Base"
+ },
+ {
+ "name": "HIP 53688",
+ "pos_x": -6.15625,
+ "pos_y": 137.1875,
+ "pos_z": -67.5625,
+ "stations": "White Gateway,Samos' Progress,Hurston Depot"
+ },
+ {
+ "name": "HIP 53699",
+ "pos_x": 143.53125,
+ "pos_y": 63.21875,
+ "pos_z": 20.90625,
+ "stations": "Guidoni Base,Voss Orbital,Godel Landing"
+ },
+ {
+ "name": "HIP 53719",
+ "pos_x": 118.0625,
+ "pos_y": 6.125,
+ "pos_z": 39.09375,
+ "stations": "Pinzon Ring,Iqbal Station,Quick Orbital,Smith City,Wetherbee Depot,Akers Holdings,Birmingham Works,Hovell Prospect"
+ },
+ {
+ "name": "HIP 53737",
+ "pos_x": 64.53125,
+ "pos_y": 130.09375,
+ "pos_z": -38.65625,
+ "stations": "Geston Enterprise"
+ },
+ {
+ "name": "HIP 53756",
+ "pos_x": -10.5,
+ "pos_y": 121.03125,
+ "pos_z": -61,
+ "stations": "Jordan City,Clute Terminal,Stjepan Seljan Gateway,Burkin Hub,Lanier Camp,Oltion Hub,Rangarajan Port,Patterson Terminal,Rayhan al-Biruni Ring"
+ },
+ {
+ "name": "HIP 5381",
+ "pos_x": 103.28125,
+ "pos_y": -184.8125,
+ "pos_z": 55.65625,
+ "stations": "Whelan Camp"
+ },
+ {
+ "name": "HIP 53879",
+ "pos_x": 110.84375,
+ "pos_y": 102.53125,
+ "pos_z": -7.8125,
+ "stations": "Rukavishnikov Terminal,Moseley Enterprise,Koch Point,Beadle Station,Pogue Survey"
+ },
+ {
+ "name": "HIP 53898",
+ "pos_x": 40.25,
+ "pos_y": 126.875,
+ "pos_z": -44.46875,
+ "stations": "Fowler Enterprise,Holdstock Palace,MacLeod Depot"
+ },
+ {
+ "name": "HIP 53923",
+ "pos_x": 43.34375,
+ "pos_y": 152.53125,
+ "pos_z": -55,
+ "stations": "Hodgson Dock,Womack Gateway,Przhevalsky Port,Liouville Base,Roberts Installation"
+ },
+ {
+ "name": "HIP 54040",
+ "pos_x": -37.53125,
+ "pos_y": 118.28125,
+ "pos_z": -67.875,
+ "stations": "Nesvadba Dock,Kelly Depot,Utley Prospect"
+ },
+ {
+ "name": "HIP 54047",
+ "pos_x": 0.125,
+ "pos_y": 159.84375,
+ "pos_z": -73,
+ "stations": "Wallis Outpost"
+ },
+ {
+ "name": "HIP 54072",
+ "pos_x": 106.96875,
+ "pos_y": 123.53125,
+ "pos_z": -16.96875,
+ "stations": "Alenquer Settlement,Asher Base,Rosenberg Laboratory"
+ },
+ {
+ "name": "HIP 54083",
+ "pos_x": 146.5,
+ "pos_y": -37.9375,
+ "pos_z": 71.1875,
+ "stations": "Stefansson Vision,Loewy Installation,Crossfield Hub,Hind Mines"
+ },
+ {
+ "name": "HIP 54210",
+ "pos_x": -0.3125,
+ "pos_y": 130.09375,
+ "pos_z": -58.4375,
+ "stations": "Chebyshev Platform,Barcelos Works,Davidson Point"
+ },
+ {
+ "name": "HIP 54233",
+ "pos_x": -25.4375,
+ "pos_y": 111.40625,
+ "pos_z": -59.34375,
+ "stations": "Berezin Terminal,Morgan Beacon,Santos Installation"
+ },
+ {
+ "name": "HIP 54346",
+ "pos_x": 73.96875,
+ "pos_y": 142.53125,
+ "pos_z": -35.09375,
+ "stations": "Kozlov Dock,Bernoulli Horizons"
+ },
+ {
+ "name": "HIP 54362",
+ "pos_x": 127.0625,
+ "pos_y": 65.25,
+ "pos_z": 19.15625,
+ "stations": "Knapp Station,Baffin Bastion"
+ },
+ {
+ "name": "HIP 54366",
+ "pos_x": 126.25,
+ "pos_y": 108.375,
+ "pos_z": -0.125,
+ "stations": "Cernan Dock"
+ },
+ {
+ "name": "HIP 54405",
+ "pos_x": 145.46875,
+ "pos_y": -12.1875,
+ "pos_z": 60.4375,
+ "stations": "Helmholtz Colony,Isherwood Plant,Avogadro Depot,Bacon Survey,Patsayev Hub"
+ },
+ {
+ "name": "HIP 54422",
+ "pos_x": 2.84375,
+ "pos_y": 132.78125,
+ "pos_z": -56.9375,
+ "stations": "Sagan Outpost,Reamy Landing"
+ },
+ {
+ "name": "HIP 5443",
+ "pos_x": -29.46875,
+ "pos_y": -114.84375,
+ "pos_z": -32.21875,
+ "stations": "Alvarez de Pineda Hub,Apianus Mines,Belyavsky Works"
+ },
+ {
+ "name": "HIP 54530",
+ "pos_x": 110.9375,
+ "pos_y": 68.40625,
+ "pos_z": 12.96875,
+ "stations": "Northrop Enterprise,Dobrovolskiy Terminal,Pettit Dock,Mukai Beacon,Szebehely Survey"
+ },
+ {
+ "name": "HIP 54536",
+ "pos_x": -8.40625,
+ "pos_y": 114.59375,
+ "pos_z": -52.59375,
+ "stations": "Person Port,Gabriel Dock,Griffith Station,Kafka Installation,Elder Orbital,Shalatula Lab,Nordenskiold Survey"
+ },
+ {
+ "name": "HIP 54614",
+ "pos_x": -9.25,
+ "pos_y": 110.28125,
+ "pos_z": -50.59375,
+ "stations": "Ziemkiewicz Gateway,Denton Keep,McBride Landing"
+ },
+ {
+ "name": "HIP 54641",
+ "pos_x": 162.90625,
+ "pos_y": -14.6875,
+ "pos_z": 69.25,
+ "stations": "Linaweaver Platform,O'Brien Enterprise"
+ },
+ {
+ "name": "HIP 54642",
+ "pos_x": 148.09375,
+ "pos_y": 63.96875,
+ "pos_z": 30.15625,
+ "stations": "Laliberte Landing,Koch Platform"
+ },
+ {
+ "name": "HIP 54681",
+ "pos_x": -13.1875,
+ "pos_y": 143.09375,
+ "pos_z": -65.53125,
+ "stations": "Blackman Dock"
+ },
+ {
+ "name": "HIP 54690",
+ "pos_x": 135.1875,
+ "pos_y": 74.53125,
+ "pos_z": 21.1875,
+ "stations": "Dorsey Outpost,Szameit Legacy,Maddox Beacon"
+ },
+ {
+ "name": "HIP 54692",
+ "pos_x": -12.8125,
+ "pos_y": 138.25,
+ "pos_z": -63.21875,
+ "stations": "Brust Vision,Pellegrino Settlement,Weyl Colony"
+ },
+ {
+ "name": "HIP 54697",
+ "pos_x": 36.625,
+ "pos_y": 111,
+ "pos_z": -32.46875,
+ "stations": "Davy Terminal,Allen Beacon"
+ },
+ {
+ "name": "HIP 54844",
+ "pos_x": 50.09375,
+ "pos_y": 163.65625,
+ "pos_z": -47.875,
+ "stations": "Abnett Base"
+ },
+ {
+ "name": "HIP 54846",
+ "pos_x": 143.25,
+ "pos_y": -7.4375,
+ "pos_z": 59.53125,
+ "stations": "Virtanen Station,Menzies Terminal,Mills Port"
+ },
+ {
+ "name": "HIP 5488",
+ "pos_x": 122.28125,
+ "pos_y": -240.90625,
+ "pos_z": 62.65625,
+ "stations": "Smoot Vision"
+ },
+ {
+ "name": "HIP 54977",
+ "pos_x": 170.4375,
+ "pos_y": 22.84375,
+ "pos_z": 58.6875,
+ "stations": "Nachtigal Arsenal"
+ },
+ {
+ "name": "HIP 54984",
+ "pos_x": 149.96875,
+ "pos_y": 46.78125,
+ "pos_z": 40.875,
+ "stations": "Bombelli Bastion"
+ },
+ {
+ "name": "HIP 55109",
+ "pos_x": 164.53125,
+ "pos_y": 16.75,
+ "pos_z": 59.59375,
+ "stations": "Ross Base,Gaiman Settlement,Mille Enterprise"
+ },
+ {
+ "name": "HIP 55118",
+ "pos_x": 35.46875,
+ "pos_y": 127.375,
+ "pos_z": -36.46875,
+ "stations": "Drzewiecki Market,Julian Enterprise,Lopez de Villalobos Enterprise,Bogdanov Ring,Hermite Port,Roberts City,Gunn Hub"
+ },
+ {
+ "name": "HIP 55159",
+ "pos_x": 132.15625,
+ "pos_y": 51.8125,
+ "pos_z": 32.90625,
+ "stations": "Tull Stop"
+ },
+ {
+ "name": "HIP 55235",
+ "pos_x": 96.8125,
+ "pos_y": 102.25,
+ "pos_z": -0.625,
+ "stations": "Wandrei Beacon,Panshin Hangar"
+ },
+ {
+ "name": "HIP 55251",
+ "pos_x": 2.53125,
+ "pos_y": 112.25,
+ "pos_z": -42.8125,
+ "stations": "Nielsen Refinery"
+ },
+ {
+ "name": "HIP 55262",
+ "pos_x": 54.125,
+ "pos_y": 129.90625,
+ "pos_z": -28.53125,
+ "stations": "Kozeyev Refinery,Kwolek Relay,Hawley Silo,Murray Lab"
+ },
+ {
+ "name": "HIP 5527",
+ "pos_x": -77.71875,
+ "pos_y": -129.3125,
+ "pos_z": -68.875,
+ "stations": "Snyder Platform"
+ },
+ {
+ "name": "HIP 55288",
+ "pos_x": 91.90625,
+ "pos_y": 126.6875,
+ "pos_z": -11.625,
+ "stations": "Longyear Orbital,Goonan Port,Chu's Folly"
+ },
+ {
+ "name": "HIP 55455",
+ "pos_x": 71.59375,
+ "pos_y": 132.375,
+ "pos_z": -20.59375,
+ "stations": "Thornton Survey"
+ },
+ {
+ "name": "HIP 55459",
+ "pos_x": -44.625,
+ "pos_y": 107.53125,
+ "pos_z": -59.34375,
+ "stations": "Kennedy Port,Maybury Horizons,Abe Bastion,Herjulfsson Orbital"
+ },
+ {
+ "name": "HIP 55469",
+ "pos_x": 166.3125,
+ "pos_y": 88.53125,
+ "pos_z": 35.46875,
+ "stations": "Shaikh Oasis,Nachtigal Oasis,He Holdings,Burgess Depot"
+ },
+ {
+ "name": "HIP 5549",
+ "pos_x": 175.65625,
+ "pos_y": -144.25,
+ "pos_z": 110.28125,
+ "stations": "Baracchi Landing,Becvar Dock,Alden Legacy,Roelofs Beacon"
+ },
+ {
+ "name": "HIP 55638",
+ "pos_x": 64.875,
+ "pos_y": 108.875,
+ "pos_z": -12.90625,
+ "stations": "Spring City,Reiter Landing,Rushd Depot"
+ },
+ {
+ "name": "HIP 55666",
+ "pos_x": 102.25,
+ "pos_y": 125.9375,
+ "pos_z": -3.0625,
+ "stations": "Sellings Colony,Andrews Orbital,Arthur Terminal,Larbalestier Point"
+ },
+ {
+ "name": "HIP 55714",
+ "pos_x": 133.6875,
+ "pos_y": 70.875,
+ "pos_z": 30.84375,
+ "stations": "Littlewood Port,Citi Vision,Dayuan Silo"
+ },
+ {
+ "name": "HIP 55761",
+ "pos_x": -13.6875,
+ "pos_y": 131.03125,
+ "pos_z": -53.3125,
+ "stations": "Cayley Hub,Oliver Terminal,Felice Dock"
+ },
+ {
+ "name": "HIP 5578",
+ "pos_x": -20.84375,
+ "pos_y": -127.9375,
+ "pos_z": -28.6875,
+ "stations": "Ejeta Depot"
+ },
+ {
+ "name": "HIP 55823",
+ "pos_x": 39.4375,
+ "pos_y": 144.125,
+ "pos_z": -34.78125,
+ "stations": "Rucker's Folly,Abbott Observatory,Bacigalupi Hub"
+ },
+ {
+ "name": "HIP 56092",
+ "pos_x": -37.34375,
+ "pos_y": 111.46875,
+ "pos_z": -54.65625,
+ "stations": "Brothers City,Csoma Port,Birmingham Terminal,Sauma Dock,Garnier Enterprise,Leichhardt Prospect,Dummer Base,Wait Colburn Survey"
+ },
+ {
+ "name": "HIP 56145",
+ "pos_x": -16.78125,
+ "pos_y": 131.75,
+ "pos_z": -52.3125,
+ "stations": "Stevin Hub,Bass Hub,Burckhardt Dock,Crown Station,Sinisalo Terminal,Burgess' Progress,Alvarez de Pineda Settlement,Piserchia Vision,Budrys Base,Lerner Keep,Bunnell Port"
+ },
+ {
+ "name": "HIP 56177",
+ "pos_x": -19.0625,
+ "pos_y": 145.5,
+ "pos_z": -57.78125,
+ "stations": "Baffin Relay,Hill Survey"
+ },
+ {
+ "name": "HIP 56213",
+ "pos_x": 97.59375,
+ "pos_y": 108.25,
+ "pos_z": 6.53125,
+ "stations": "Conway Survey,Cayley Settlement"
+ },
+ {
+ "name": "HIP 56229",
+ "pos_x": -10.15625,
+ "pos_y": 136.5625,
+ "pos_z": -50.4375,
+ "stations": "Navigator Enterprise,Kazantsev Settlement"
+ },
+ {
+ "name": "HIP 5623",
+ "pos_x": 131.34375,
+ "pos_y": -144.59375,
+ "pos_z": 78.25,
+ "stations": "Gunter Station,d'Arrest Ring,Stross Installation,Temple Works"
+ },
+ {
+ "name": "HIP 56278",
+ "pos_x": 147.53125,
+ "pos_y": 82.46875,
+ "pos_z": 37.84375,
+ "stations": "Malenchenko Dock,Bacon Dock,Crippen Platform,Tavernier Barracks"
+ },
+ {
+ "name": "HIP 56321",
+ "pos_x": 44.25,
+ "pos_y": 145.5625,
+ "pos_z": -28.5625,
+ "stations": "Otto Point,Evans Reach"
+ },
+ {
+ "name": "HIP 56337",
+ "pos_x": -37.40625,
+ "pos_y": 125.5,
+ "pos_z": -58.15625,
+ "stations": "Elgin Landing,Caillie Landing,Clairaut Base"
+ },
+ {
+ "name": "HIP 56447",
+ "pos_x": 162.3125,
+ "pos_y": 42.21875,
+ "pos_z": 59.125,
+ "stations": "Seega Dock,Chaudhary Colony"
+ },
+ {
+ "name": "HIP 56501",
+ "pos_x": 146.5,
+ "pos_y": 17.28125,
+ "pos_z": 60.40625,
+ "stations": "H. G. Wells Relay,Yolen Relay,Porges Bastion"
+ },
+ {
+ "name": "HIP 5651",
+ "pos_x": -8.84375,
+ "pos_y": -185.6875,
+ "pos_z": -27.3125,
+ "stations": "Gabrielli Platform,Gould Station"
+ },
+ {
+ "name": "HIP 56519",
+ "pos_x": 162.25,
+ "pos_y": 1.34375,
+ "pos_z": 72.75,
+ "stations": "Tapinas' Progress,Perez Landing"
+ },
+ {
+ "name": "HIP 56572",
+ "pos_x": 96.59375,
+ "pos_y": 129.71875,
+ "pos_z": 2.40625,
+ "stations": "Obruchev Landing,Burstein Sanctuary"
+ },
+ {
+ "name": "HIP 5661",
+ "pos_x": 44.875,
+ "pos_y": -221.75,
+ "pos_z": 7.09375,
+ "stations": "Boscovich Station,Fehrenbach Vision,Phillips Prospect,Rodrigues Camp"
+ },
+ {
+ "name": "HIP 56641",
+ "pos_x": 107.28125,
+ "pos_y": 114.125,
+ "pos_z": 12.9375,
+ "stations": "Hovgaard Gateway,Snodgrass Port,Gaiman Port,Brosnan Base,Fremion Landing"
+ },
+ {
+ "name": "HIP 56715",
+ "pos_x": 114.5,
+ "pos_y": 26.78125,
+ "pos_z": 44.0625,
+ "stations": "Mitra Prospect,Soukup Station,Payne Landing"
+ },
+ {
+ "name": "HIP 56791",
+ "pos_x": 137.59375,
+ "pos_y": 128.59375,
+ "pos_z": 23.78125,
+ "stations": "Lopez de Haro Survey"
+ },
+ {
+ "name": "HIP 5687",
+ "pos_x": -80.40625,
+ "pos_y": -97.3125,
+ "pos_z": -69.21875,
+ "stations": "Stefanyshyn-Piper Camp"
+ },
+ {
+ "name": "HIP 56905",
+ "pos_x": -48.15625,
+ "pos_y": 105.8125,
+ "pos_z": -54.1875,
+ "stations": "Silves Enterprise,Wandrei City,Gessi Port,Cartan Enterprise,Cowper Hub,Verrazzano Terminal,Burkin Station,McIntyre Hub,Vasilyev Relay,Britnev Holdings"
+ },
+ {
+ "name": "HIP 56930",
+ "pos_x": 56.65625,
+ "pos_y": 129.5,
+ "pos_z": -12.5,
+ "stations": "Pudwill Gorie Platform,Morgan Holdings,Schlegel Station"
+ },
+ {
+ "name": "HIP 56950",
+ "pos_x": 130.6875,
+ "pos_y": -7.21875,
+ "pos_z": 62.875,
+ "stations": "Wingqvist Orbital,Cowper Hub,McDivitt Enterprise,Rawat Keep"
+ },
+ {
+ "name": "HIP 56955",
+ "pos_x": 65.90625,
+ "pos_y": 120.15625,
+ "pos_z": -5.25,
+ "stations": "Steinmuller Dock,Heaviside Dock,Valdes Dock,Bendell Survey,Constantine Observatory"
+ },
+ {
+ "name": "HIP 56960",
+ "pos_x": 80.78125,
+ "pos_y": 98.6875,
+ "pos_z": 8.125,
+ "stations": "Veach Hub,Janes Survey,He Plant,Lavrador Enterprise,Payne Plant,RenenBellot Arsenal,Brunner Stop"
+ },
+ {
+ "name": "HIP 5700",
+ "pos_x": 133.25,
+ "pos_y": -144.6875,
+ "pos_z": 79.25,
+ "stations": "Staus City,Bracewell Port,Wallis Lab,Kopal Terminal,Halley Relay"
+ },
+ {
+ "name": "HIP 57059",
+ "pos_x": 139.8125,
+ "pos_y": 54.71875,
+ "pos_z": 49.4375,
+ "stations": "Alvares Sanctuary"
+ },
+ {
+ "name": "HIP 57080",
+ "pos_x": 54.3125,
+ "pos_y": 141.71875,
+ "pos_z": -15.9375,
+ "stations": "Frimout Platform,Sharman Platform,Laird Prospect"
+ },
+ {
+ "name": "HIP 57112",
+ "pos_x": 26.65625,
+ "pos_y": 143.78125,
+ "pos_z": -29.21875,
+ "stations": "Nicholson Horizons"
+ },
+ {
+ "name": "HIP 5712",
+ "pos_x": 116.5,
+ "pos_y": -201.34375,
+ "pos_z": 60.5,
+ "stations": "Herzog Station,Urata Survey,d'Arrest Landing,Hippalus Installation"
+ },
+ {
+ "name": "HIP 57181",
+ "pos_x": 117,
+ "pos_y": 121.5,
+ "pos_z": 20.59375,
+ "stations": "Jiushao Terminal,Kroehl Port,Morris Base,Williams Installation"
+ },
+ {
+ "name": "HIP 57269",
+ "pos_x": 143.34375,
+ "pos_y": 32.53125,
+ "pos_z": 59.03125,
+ "stations": "Bursch Terminal"
+ },
+ {
+ "name": "HIP 57346",
+ "pos_x": -20.5,
+ "pos_y": 124.03125,
+ "pos_z": -44.25,
+ "stations": "Bester Hangar,Riemann Orbital"
+ },
+ {
+ "name": "HIP 57349",
+ "pos_x": -32.1875,
+ "pos_y": 147.125,
+ "pos_z": -56.21875,
+ "stations": "Buckell Port,Mitra Gateway,Giles Enterprise,Schweickart Terminal,Celsius Barracks"
+ },
+ {
+ "name": "HIP 57350",
+ "pos_x": -59.5625,
+ "pos_y": 99.46875,
+ "pos_z": -56.0625,
+ "stations": "Knapp Enterprise,Parazynski City,Spassky Keep"
+ },
+ {
+ "name": "HIP 57352",
+ "pos_x": 135.53125,
+ "pos_y": -25.0625,
+ "pos_z": 71.71875,
+ "stations": "Chaudhary Terminal,Fernandes Barracks,Lonchakov Market"
+ },
+ {
+ "name": "HIP 57402",
+ "pos_x": 141.5,
+ "pos_y": 63.1875,
+ "pos_z": 50.46875,
+ "stations": "Underwood Camp,Hernandez Enterprise,Chilton Terminal,Slade Hub"
+ },
+ {
+ "name": "HIP 57532",
+ "pos_x": -112.3125,
+ "pos_y": 117.9375,
+ "pos_z": -86,
+ "stations": "Hand Settlement,Hendel Holdings,Maddox Relay"
+ },
+ {
+ "name": "HIP 57589",
+ "pos_x": 141.4375,
+ "pos_y": 65.65625,
+ "pos_z": 51.25,
+ "stations": "Cavendish Terminal,Ziemkiewicz Dock,Siodmak Port,Flinders Point,Cortes Beacon"
+ },
+ {
+ "name": "HIP 5764",
+ "pos_x": -31.53125,
+ "pos_y": -214.53125,
+ "pos_z": -48.5625,
+ "stations": "Arkhangelsky Camp,Fanning Camp"
+ },
+ {
+ "name": "HIP 57645",
+ "pos_x": 93.84375,
+ "pos_y": 81.6875,
+ "pos_z": 24.375,
+ "stations": "Spassky Enterprise"
+ },
+ {
+ "name": "HIP 5796",
+ "pos_x": 94.78125,
+ "pos_y": -238.03125,
+ "pos_z": 39.71875,
+ "stations": "Wendelin Dock,Tuttle Vision,Lagadha Beacon"
+ },
+ {
+ "name": "HIP 57984",
+ "pos_x": -41.15625,
+ "pos_y": 156.0625,
+ "pos_z": -58.46875,
+ "stations": "Clough Arsenal,Pennington Terminal"
+ },
+ {
+ "name": "HIP 58019",
+ "pos_x": 10.6875,
+ "pos_y": 146.3125,
+ "pos_z": -29.96875,
+ "stations": "Glazkov Point,Stephenson Base"
+ },
+ {
+ "name": "HIP 58029",
+ "pos_x": 118.4375,
+ "pos_y": 75.71875,
+ "pos_z": 41,
+ "stations": "Gagnan Hub,Smeaton Beacon"
+ },
+ {
+ "name": "HIP 58056",
+ "pos_x": 39.28125,
+ "pos_y": 129.1875,
+ "pos_z": -11.28125,
+ "stations": "Walker Installation,Viehbock Terminal,Parmitano Settlement"
+ },
+ {
+ "name": "HIP 58083",
+ "pos_x": 59.78125,
+ "pos_y": 114.90625,
+ "pos_z": 2.625,
+ "stations": "Rasch Enterprise,Fisk Orbital,Zindell Hub,Davis Base,Ziemianski Hub,Fadlan Arena,Pytheas Terminal,Heyerdahl Keep,Wilson Beacon"
+ },
+ {
+ "name": "HIP 58085",
+ "pos_x": 125.46875,
+ "pos_y": -10.78125,
+ "pos_z": 65.5625,
+ "stations": "Redi Mine,Ibold Settlement,Hilmers' Claim"
+ },
+ {
+ "name": "HIP 58180",
+ "pos_x": 132.75,
+ "pos_y": -40.28125,
+ "pos_z": 76.46875,
+ "stations": "Marius Orbital,Nadiradze Laboratory,Abetti Gateway"
+ },
+ {
+ "name": "HIP 58213",
+ "pos_x": 23.28125,
+ "pos_y": 203.125,
+ "pos_z": -35.21875,
+ "stations": "Santos' Claim"
+ },
+ {
+ "name": "HIP 58237",
+ "pos_x": 72.5,
+ "pos_y": 114.09375,
+ "pos_z": 10.53125,
+ "stations": "Hardy's Folly,Goldstein Prison"
+ },
+ {
+ "name": "HIP 58268",
+ "pos_x": 84.65625,
+ "pos_y": 124.78125,
+ "pos_z": 14.46875,
+ "stations": "Person Holdings,Dupuy de Lome Enterprise,Sekelj Settlement"
+ },
+ {
+ "name": "HIP 58287",
+ "pos_x": -15.0625,
+ "pos_y": 140.65625,
+ "pos_z": -39.6875,
+ "stations": "Clayton Survey,Birmingham Depot,Malcolm Survey"
+ },
+ {
+ "name": "HIP 58301",
+ "pos_x": 164.875,
+ "pos_y": 39.75,
+ "pos_z": 74.875,
+ "stations": "Karlsefni Port,Gaspar de Portola Platform,Sharp Enterprise"
+ },
+ {
+ "name": "HIP 58315",
+ "pos_x": 131.46875,
+ "pos_y": 58.28125,
+ "pos_z": 53.78125,
+ "stations": "Offutt City,Werber Orbital,Makarov Penal colony"
+ },
+ {
+ "name": "HIP 58317",
+ "pos_x": 136.34375,
+ "pos_y": 97.90625,
+ "pos_z": 47.28125,
+ "stations": "Baraniecki Port,von Bellingshausen Survey,Abbott Hub,Holdstock Beacon"
+ },
+ {
+ "name": "HIP 5840",
+ "pos_x": -17.78125,
+ "pos_y": -161.875,
+ "pos_z": -33.0625,
+ "stations": "Herzog Prospect,Lozino-Lozinskiy Port,Stephenson Vision"
+ },
+ {
+ "name": "HIP 58412",
+ "pos_x": -51.03125,
+ "pos_y": 109.0625,
+ "pos_z": -50.34375,
+ "stations": "Pawelczyk Dock,Knapp Gateway,Abasheli Gateway"
+ },
+ {
+ "name": "HIP 5853",
+ "pos_x": -11.8125,
+ "pos_y": -223.25,
+ "pos_z": -36.53125,
+ "stations": "Hornoch Hub,Bartini Enterprise,Davis Penal colony,Beliaev Silo"
+ },
+ {
+ "name": "HIP 58577",
+ "pos_x": 13.3125,
+ "pos_y": 199.375,
+ "pos_z": -35.46875,
+ "stations": "Cayley Colony,North Installation,O'Donnell Point"
+ },
+ {
+ "name": "HIP 5881",
+ "pos_x": -110.28125,
+ "pos_y": -64.625,
+ "pos_z": -88.53125,
+ "stations": "Kaleri Port,Eisenstein Settlement"
+ },
+ {
+ "name": "HIP 58815",
+ "pos_x": 126.28125,
+ "pos_y": 44.375,
+ "pos_z": 57.5625,
+ "stations": "Eisele Enterprise,Still Enterprise,Mendez Ring,Ramsay Enterprise,Thomas Port,Kekule Gateway,Stirling Base"
+ },
+ {
+ "name": "HIP 58876",
+ "pos_x": -72.375,
+ "pos_y": 114.375,
+ "pos_z": -60.6875,
+ "stations": "Dann Station,Cooper Terminal,Langford Point"
+ },
+ {
+ "name": "HIP 58924",
+ "pos_x": -115.6875,
+ "pos_y": 121.9375,
+ "pos_z": -84.9375,
+ "stations": "Shunn City,Fast Dock,Dampier Dock,Nansen Base,Busch Relay,Weber's Inheritance"
+ },
+ {
+ "name": "HIP 58945",
+ "pos_x": 43.1875,
+ "pos_y": 130.25,
+ "pos_z": -2.34375,
+ "stations": "Hoffman Base,Garfinkle Dock,Svavarsson Installation,Jordan Platform,Tolstoi Lab"
+ },
+ {
+ "name": "HIP 58986",
+ "pos_x": 77.0625,
+ "pos_y": 118.28125,
+ "pos_z": 18.21875,
+ "stations": "Coulomb Dock,Smith Vision"
+ },
+ {
+ "name": "HIP 59024",
+ "pos_x": -43.46875,
+ "pos_y": 160.28125,
+ "pos_z": -53.5625,
+ "stations": "Clifford Station,Musgrave Holdings,Marques Barracks"
+ },
+ {
+ "name": "HIP 59077",
+ "pos_x": 140.25,
+ "pos_y": 67.53125,
+ "pos_z": 62.34375,
+ "stations": "Rochon Landing,Williams Station,Soper Prospect"
+ },
+ {
+ "name": "HIP 59143",
+ "pos_x": 94.4375,
+ "pos_y": 78.125,
+ "pos_z": 36.34375,
+ "stations": "Hopkins Ring,Houssay Orbital,McMonagle Hub,Janes Keep,Hartsfield Station,Napier Orbital,Chretien Gateway"
+ },
+ {
+ "name": "HIP 59180",
+ "pos_x": -52,
+ "pos_y": 132.40625,
+ "pos_z": -51.90625,
+ "stations": "Cardano Port,Bombelli Dock,Reed Penal colony"
+ },
+ {
+ "name": "HIP 59310",
+ "pos_x": 29.625,
+ "pos_y": 141.5625,
+ "pos_z": -8.375,
+ "stations": "Bayley's Progress,Pauli Bastion,Younghusband Refinery"
+ },
+ {
+ "name": "HIP 59315",
+ "pos_x": 107.03125,
+ "pos_y": 27.4375,
+ "pos_z": 53.34375,
+ "stations": "Fullerton Port,Drebbel Base"
+ },
+ {
+ "name": "HIP 59417",
+ "pos_x": 89.15625,
+ "pos_y": 134.90625,
+ "pos_z": 26.0625,
+ "stations": "Crown Landing,Wood Depot,Lawson Settlement"
+ },
+ {
+ "name": "HIP 59419",
+ "pos_x": 124.125,
+ "pos_y": 42.8125,
+ "pos_z": 60.59375,
+ "stations": "Bykovsky Camp"
+ },
+ {
+ "name": "HIP 59422",
+ "pos_x": 83.90625,
+ "pos_y": 136.6875,
+ "pos_z": 22.96875,
+ "stations": "Clark Mines,Farmer Survey,Mitchell Vision"
+ },
+ {
+ "name": "HIP 59486",
+ "pos_x": 19.625,
+ "pos_y": 139.59375,
+ "pos_z": -12.21875,
+ "stations": "Steakley Dock,Elcano Dock"
+ },
+ {
+ "name": "HIP 59533",
+ "pos_x": 122.96875,
+ "pos_y": -9.0625,
+ "pos_z": 69,
+ "stations": "Burnham Beacon,Heyerdahl Installation"
+ },
+ {
+ "name": "HIP 596",
+ "pos_x": 160.1875,
+ "pos_y": -168.90625,
+ "pos_z": 116.9375,
+ "stations": "Weaver Dock,Baracchi Prospect,Cogswell Relay"
+ },
+ {
+ "name": "HIP 59610",
+ "pos_x": 40.375,
+ "pos_y": 116.09375,
+ "pos_z": 3.9375,
+ "stations": "McIntyre Station"
+ },
+ {
+ "name": "HIP 59655",
+ "pos_x": 58.5,
+ "pos_y": 134.5625,
+ "pos_z": 11.4375,
+ "stations": "Moseley Settlement,Nye Dock"
+ },
+ {
+ "name": "HIP 59735",
+ "pos_x": 4.5,
+ "pos_y": 173.65625,
+ "pos_z": -23.78125,
+ "stations": "Carstensz Platform,Niven's Inheritance"
+ },
+ {
+ "name": "HIP 59772",
+ "pos_x": -11.34375,
+ "pos_y": 127.75,
+ "pos_z": -25.46875,
+ "stations": "Covey Orbital,Peterson City,Robinson Depot"
+ },
+ {
+ "name": "HIP 59776",
+ "pos_x": -17.03125,
+ "pos_y": 129.5625,
+ "pos_z": -28.875,
+ "stations": "O'Brien Ring,Williams Installation,Alten Installation,Lavrador Base"
+ },
+ {
+ "name": "HIP 59846",
+ "pos_x": -82.125,
+ "pos_y": 165.0625,
+ "pos_z": -70,
+ "stations": "Brust Survey,Wiener Enterprise"
+ },
+ {
+ "name": "HIP 59879",
+ "pos_x": -92.15625,
+ "pos_y": 66.25,
+ "pos_z": -61.1875,
+ "stations": "Samuda Station,Garfinkle Terminal,Gaultier de Varennes Terminal,Alexandria Hub,Kafka Dock,Desargues Dock,Caillie Ring"
+ },
+ {
+ "name": "HIP 60016",
+ "pos_x": 129.40625,
+ "pos_y": 52.34375,
+ "pos_z": 66,
+ "stations": "Russell Oasis,Chandler Prospect"
+ },
+ {
+ "name": "HIP 60051",
+ "pos_x": 155.40625,
+ "pos_y": 51.59375,
+ "pos_z": 81.0625,
+ "stations": "Smith Settlement"
+ },
+ {
+ "name": "HIP 60061",
+ "pos_x": 10.03125,
+ "pos_y": 198.25,
+ "pos_z": -20.90625,
+ "stations": "Hughes Port,Capek Horizons,Watts Landing"
+ },
+ {
+ "name": "HIP 6011",
+ "pos_x": 33,
+ "pos_y": -199.09375,
+ "pos_z": -2.9375,
+ "stations": "Fedden Terminal,Jones Terminal,Michell Station,Fabian Port,Bonestell Vision,Dubyago Port,Kepler Station,i Sola Hub,Pearse Port,Slusser Vision,Muramatsu Installation,Drake Depot,Gibbs Depot,Janszoon Beacon"
+ },
+ {
+ "name": "HIP 60149",
+ "pos_x": 162.78125,
+ "pos_y": 82.84375,
+ "pos_z": 81.96875,
+ "stations": "Noli Camp,Milnor Depot,Bishop's Progress"
+ },
+ {
+ "name": "HIP 60174",
+ "pos_x": 74.78125,
+ "pos_y": 131.15625,
+ "pos_z": 25.8125,
+ "stations": "Auer Settlement,Godwin Dock,Jeschke Barracks"
+ },
+ {
+ "name": "HIP 60186",
+ "pos_x": 0.0625,
+ "pos_y": 143.78125,
+ "pos_z": -18.28125,
+ "stations": "Greenleaf Landing"
+ },
+ {
+ "name": "HIP 60230",
+ "pos_x": 14.75,
+ "pos_y": 159.09375,
+ "pos_z": -11.46875,
+ "stations": "Rucker Hangar"
+ },
+ {
+ "name": "HIP 6024",
+ "pos_x": 127.15625,
+ "pos_y": -207.71875,
+ "pos_z": 64.90625,
+ "stations": "Gibbs Enterprise,Shapley Holdings,Peters Holdings,Porco Holdings"
+ },
+ {
+ "name": "HIP 60265",
+ "pos_x": -5.5625,
+ "pos_y": 120.9375,
+ "pos_z": -18.125,
+ "stations": "Reed Gateway,Peral Vision,Kiernan Enterprise,Chios Landing,Wandrei Point,Jones City"
+ },
+ {
+ "name": "HIP 60321",
+ "pos_x": 145.375,
+ "pos_y": 11.59375,
+ "pos_z": 82.1875,
+ "stations": "Coblentz Terminal,Lee Port,Grothendieck Hub"
+ },
+ {
+ "name": "HIP 60325",
+ "pos_x": 41.4375,
+ "pos_y": 137.3125,
+ "pos_z": 7.28125,
+ "stations": "McCaffrey Vision,Griffin Terminal"
+ },
+ {
+ "name": "HIP 60346",
+ "pos_x": -3.25,
+ "pos_y": 126.3125,
+ "pos_z": -16.9375,
+ "stations": "Archer Dock"
+ },
+ {
+ "name": "HIP 60422",
+ "pos_x": 123.78125,
+ "pos_y": 71.625,
+ "pos_z": 63.3125,
+ "stations": "Felice Hub,Hovgaard Platform,Cabral Keep,Martins Camp"
+ },
+ {
+ "name": "HIP 60501",
+ "pos_x": 59.59375,
+ "pos_y": 139.25,
+ "pos_z": 19.09375,
+ "stations": "MacLeod Vision"
+ },
+ {
+ "name": "HIP 60541",
+ "pos_x": 63.78125,
+ "pos_y": 156.71875,
+ "pos_z": 19.96875,
+ "stations": "Vizcaino Colony"
+ },
+ {
+ "name": "HIP 60551",
+ "pos_x": -15.625,
+ "pos_y": 132.125,
+ "pos_z": -23.46875,
+ "stations": "Baturin City,Pudwill Gorie Hub,Lovelace Station,Howard Orbital,Carr Orbital,Shepherd Vision"
+ },
+ {
+ "name": "HIP 60588",
+ "pos_x": -59.875,
+ "pos_y": 115.03125,
+ "pos_z": -47.28125,
+ "stations": "Obruchev Station,Narvaez Enterprise,Helmholtz Survey,Ahmed Settlement,Bishop Stop"
+ },
+ {
+ "name": "HIP 60614",
+ "pos_x": -14.90625,
+ "pos_y": 135.5625,
+ "pos_z": -23.09375,
+ "stations": "Thiele Enterprise"
+ },
+ {
+ "name": "HIP 60633",
+ "pos_x": 18.3125,
+ "pos_y": 138.59375,
+ "pos_z": -3.90625,
+ "stations": "McMullen Terminal,VanderMeer Plant,Zelazny Installation"
+ },
+ {
+ "name": "HIP 60648",
+ "pos_x": 55.15625,
+ "pos_y": 106.40625,
+ "pos_z": 21.1875,
+ "stations": "Descartes Ring,Francisco de Eliza Installation,Rawat Observatory"
+ },
+ {
+ "name": "HIP 60652",
+ "pos_x": -62.90625,
+ "pos_y": 113.53125,
+ "pos_z": -48.625,
+ "stations": "Hudson Ring"
+ },
+ {
+ "name": "HIP 6066",
+ "pos_x": 61.78125,
+ "pos_y": -234.53125,
+ "pos_z": 12.78125,
+ "stations": "Wood Port"
+ },
+ {
+ "name": "HIP 60662",
+ "pos_x": 140.25,
+ "pos_y": 38.75,
+ "pos_z": 78.09375,
+ "stations": "Thuot Settlement,Otiman Installation"
+ },
+ {
+ "name": "HIP 60679",
+ "pos_x": 161.0625,
+ "pos_y": 21.09375,
+ "pos_z": 92.1875,
+ "stations": "Cady Enterprise,Brera Depot,Cartan Base"
+ },
+ {
+ "name": "HIP 60788",
+ "pos_x": 136.125,
+ "pos_y": -24.9375,
+ "pos_z": 82.65625,
+ "stations": "Midgeley Terminal,Kinsey Horizons"
+ },
+ {
+ "name": "HIP 60802",
+ "pos_x": 110.65625,
+ "pos_y": 129.3125,
+ "pos_z": 52.84375,
+ "stations": "Altshuller Base"
+ },
+ {
+ "name": "HIP 60825",
+ "pos_x": 134.1875,
+ "pos_y": -17.0625,
+ "pos_z": 80.90625,
+ "stations": "Shaara Plant,Perga Installation,Smith Point,Malyshev Depot"
+ },
+ {
+ "name": "HIP 60831",
+ "pos_x": -30.84375,
+ "pos_y": 135.34375,
+ "pos_z": -31,
+ "stations": "Mendeleev Landing,Eisenstein Penal colony,Shiner Port"
+ },
+ {
+ "name": "HIP 60845",
+ "pos_x": 135.875,
+ "pos_y": 2,
+ "pos_z": 80.1875,
+ "stations": "Vaucanson Enterprise,Butz Beacon,Borman's Progress"
+ },
+ {
+ "name": "HIP 60848",
+ "pos_x": -36.59375,
+ "pos_y": 156.625,
+ "pos_z": -36.28125,
+ "stations": "Cremona Mines,Panshin Port,Harding Installation"
+ },
+ {
+ "name": "HIP 60902",
+ "pos_x": 72.25,
+ "pos_y": 154.75,
+ "pos_z": 28.8125,
+ "stations": "Ito Gateway"
+ },
+ {
+ "name": "HIP 60942",
+ "pos_x": 68.78125,
+ "pos_y": 172.6875,
+ "pos_z": 25.6875,
+ "stations": "Cochrane Colony,Ponce de Leon Vision,Blish Relay"
+ },
+ {
+ "name": "HIP 60953",
+ "pos_x": 68.53125,
+ "pos_y": 139.96875,
+ "pos_z": 28.59375,
+ "stations": "Pailes Vision,Rae Camp,Georg Bothe Dock,Jacquard Holdings,Bottego Bastion"
+ },
+ {
+ "name": "HIP 61061",
+ "pos_x": 80.25,
+ "pos_y": 170.5,
+ "pos_z": 34.09375,
+ "stations": "Lorrah Enterprise,Foden Dock,Tiptree Base"
+ },
+ {
+ "name": "HIP 61072",
+ "pos_x": -3.25,
+ "pos_y": 137.34375,
+ "pos_z": -13.125,
+ "stations": "Hill City,King Dock,Watts Station,Wingrove's Progress"
+ },
+ {
+ "name": "HIP 6108",
+ "pos_x": 0.71875,
+ "pos_y": -248.875,
+ "pos_z": -34.84375,
+ "stations": "Wheeler Hub,Bester Survey,Schmitz Silo,Longomontanus Terminal"
+ },
+ {
+ "name": "HIP 61097",
+ "pos_x": 99.84375,
+ "pos_y": 39.75,
+ "pos_z": 56.6875,
+ "stations": "Cardano Vision,Zajdel Gateway,MacLean Enterprise,Eckford Mine,Kandel Terminal"
+ },
+ {
+ "name": "HIP 61129",
+ "pos_x": 51.6875,
+ "pos_y": 171.9375,
+ "pos_z": 17.53125,
+ "stations": "Gabriel Camp"
+ },
+ {
+ "name": "HIP 61224",
+ "pos_x": 76.625,
+ "pos_y": 116.40625,
+ "pos_z": 37.6875,
+ "stations": "Cayley Orbital,Clebsch Keep,Toll Landing"
+ },
+ {
+ "name": "HIP 61272",
+ "pos_x": -11.75,
+ "pos_y": 168.75,
+ "pos_z": -19.03125,
+ "stations": "Archimedes Landing"
+ },
+ {
+ "name": "HIP 61276",
+ "pos_x": -25.6875,
+ "pos_y": 154.84375,
+ "pos_z": -26.5,
+ "stations": "Erdos Beacon,Hughes Legacy"
+ },
+ {
+ "name": "HIP 61505",
+ "pos_x": 125.9375,
+ "pos_y": 52.28125,
+ "pos_z": 74.15625,
+ "stations": "Bluford Prospect,Lobachevsky Freeport,Lie Settlement"
+ },
+ {
+ "name": "HIP 61522",
+ "pos_x": -104.9375,
+ "pos_y": 105.375,
+ "pos_z": -70.5,
+ "stations": "Ziemkiewicz Platform,Sabine Station"
+ },
+ {
+ "name": "HIP 6161",
+ "pos_x": 152.3125,
+ "pos_y": -150.09375,
+ "pos_z": 90.78125,
+ "stations": "Hogg Station,Matthaus Olbers Dock,Wnuk-Lipinski Refinery"
+ },
+ {
+ "name": "HIP 61632",
+ "pos_x": -40.3125,
+ "pos_y": 165.90625,
+ "pos_z": -33.28125,
+ "stations": "Ericsson Enterprise,Peano Silo"
+ },
+ {
+ "name": "HIP 61687",
+ "pos_x": 64.5,
+ "pos_y": 113.5625,
+ "pos_z": 34.6875,
+ "stations": "Goulart Settlement,Hand Bastion"
+ },
+ {
+ "name": "HIP 61704",
+ "pos_x": -44.0625,
+ "pos_y": 132.1875,
+ "pos_z": -33.46875,
+ "stations": "Markham Terminal,Lukyanenko Enterprise,Lee Refinery"
+ },
+ {
+ "name": "HIP 61811",
+ "pos_x": -82.90625,
+ "pos_y": 113.59375,
+ "pos_z": -56.375,
+ "stations": "Crook Depot,Lerman Settlement,Alexandria Landing"
+ },
+ {
+ "name": "HIP 61816",
+ "pos_x": 17.84375,
+ "pos_y": 153.15625,
+ "pos_z": 4.90625,
+ "stations": "Brosnan Terminal"
+ },
+ {
+ "name": "HIP 6182",
+ "pos_x": 43.96875,
+ "pos_y": -262.09375,
+ "pos_z": -5.90625,
+ "stations": "Dalgarno Landing,Neujmin Prospect,Fowler Bastion"
+ },
+ {
+ "name": "HIP 61840",
+ "pos_x": 144.46875,
+ "pos_y": 39.6875,
+ "pos_z": 88.71875,
+ "stations": "Kaleri Mines"
+ },
+ {
+ "name": "HIP 61907",
+ "pos_x": 127.375,
+ "pos_y": 39.84375,
+ "pos_z": 78.5,
+ "stations": "Anderson Dock,Chern Reformatory"
+ },
+ {
+ "name": "HIP 61947",
+ "pos_x": -26.125,
+ "pos_y": 117.53125,
+ "pos_z": -20.3125,
+ "stations": "Yano City,Tilman Port,Converse Dock,Haldeman's Progress"
+ },
+ {
+ "name": "HIP 61986",
+ "pos_x": 4.21875,
+ "pos_y": 143.46875,
+ "pos_z": -1.8125,
+ "stations": "Luiken Port,Reynolds' Inheritance"
+ },
+ {
+ "name": "HIP 62118",
+ "pos_x": 112.90625,
+ "pos_y": 78.3125,
+ "pos_z": 69.8125,
+ "stations": "Rand Mines,Sagan Outpost"
+ },
+ {
+ "name": "HIP 62124",
+ "pos_x": -12.75,
+ "pos_y": 138.09375,
+ "pos_z": -11.3125,
+ "stations": "Tshang Landing,Brothers' Folly,Alenquer Horizons"
+ },
+ {
+ "name": "HIP 62127",
+ "pos_x": 135.8125,
+ "pos_y": 83.09375,
+ "pos_z": 84.34375,
+ "stations": "Fowler Terminal,Gernsback Oasis"
+ },
+ {
+ "name": "HIP 62153",
+ "pos_x": 84.96875,
+ "pos_y": 142.03125,
+ "pos_z": 51,
+ "stations": "Heinlein Survey,Piercy Survey"
+ },
+ {
+ "name": "HIP 62160",
+ "pos_x": 60.1875,
+ "pos_y": 119.25,
+ "pos_z": 35.75,
+ "stations": "Tarelkin City,Kandel Horizons"
+ },
+ {
+ "name": "HIP 62175",
+ "pos_x": -64.90625,
+ "pos_y": 128.875,
+ "pos_z": -44,
+ "stations": "Great Point,Bethke Installation"
+ },
+ {
+ "name": "HIP 62178",
+ "pos_x": 58.875,
+ "pos_y": 153.9375,
+ "pos_z": 34.375,
+ "stations": "Burnell Orbital,Lee Terminal,Julian Orbital,Beaumont Installation"
+ },
+ {
+ "name": "HIP 62249",
+ "pos_x": 37.09375,
+ "pos_y": 183.125,
+ "pos_z": 20.6875,
+ "stations": "Atwood Relay,Lehtonen Relay"
+ },
+ {
+ "name": "HIP 62277",
+ "pos_x": 133.84375,
+ "pos_y": 34.90625,
+ "pos_z": 85.125,
+ "stations": "Popov Depot,Duke Terminal"
+ },
+ {
+ "name": "HIP 62304",
+ "pos_x": 81.75,
+ "pos_y": 128.90625,
+ "pos_z": 50.625,
+ "stations": "Schouten Port,Scalzi Prospect"
+ },
+ {
+ "name": "HIP 6234",
+ "pos_x": -14.25,
+ "pos_y": -146.5,
+ "pos_z": -32.5625,
+ "stations": "Brunel Relay,Bode Colony,Rosenberger Holdings,Verrazzano Survey"
+ },
+ {
+ "name": "HIP 62349",
+ "pos_x": 7.1875,
+ "pos_y": 135.65625,
+ "pos_z": 3.0625,
+ "stations": "Boole Port,Pryor Landing,Mitchell Orbital,Marques Arsenal"
+ },
+ {
+ "name": "HIP 62435",
+ "pos_x": 124.15625,
+ "pos_y": 67.375,
+ "pos_z": 79.8125,
+ "stations": "Clayton Legacy,Sheffield Reach"
+ },
+ {
+ "name": "HIP 62438",
+ "pos_x": 22.5625,
+ "pos_y": 178.84375,
+ "pos_z": 13.59375,
+ "stations": "Crowley Colony"
+ },
+ {
+ "name": "HIP 62454",
+ "pos_x": 8.8125,
+ "pos_y": 130.46875,
+ "pos_z": 5.0625,
+ "stations": "Wingqvist Refinery,Payne Observatory,Schmitt Station"
+ },
+ {
+ "name": "HIP 62509",
+ "pos_x": 36.15625,
+ "pos_y": 163.625,
+ "pos_z": 23.15625,
+ "stations": "Kaku Dock,Arrhenius Orbital,Bresnik Lab"
+ },
+ {
+ "name": "HIP 62536",
+ "pos_x": 27.3125,
+ "pos_y": 118.9375,
+ "pos_z": 17.65625,
+ "stations": "Onnes Orbital,Boodt Colony,Cardano Relay,Coppel Installation"
+ },
+ {
+ "name": "HIP 6254",
+ "pos_x": 79.5625,
+ "pos_y": -257.71875,
+ "pos_z": 20.125,
+ "stations": "Dhawan Station,Greenstein Vision,Daqing Station,Caryanda Terminal"
+ },
+ {
+ "name": "HIP 62540",
+ "pos_x": 46.5625,
+ "pos_y": 153.6875,
+ "pos_z": 30.15625,
+ "stations": "Eschbach Silo"
+ },
+ {
+ "name": "HIP 6262",
+ "pos_x": 86.4375,
+ "pos_y": -225.15625,
+ "pos_z": 30.0625,
+ "stations": "Kohoutek Terminal,Cannon Vision,Foster Terminal"
+ },
+ {
+ "name": "HIP 62686",
+ "pos_x": 12.03125,
+ "pos_y": 123.90625,
+ "pos_z": 8.84375,
+ "stations": "Scithers Landing"
+ },
+ {
+ "name": "HIP 62744",
+ "pos_x": 134.96875,
+ "pos_y": 41.78125,
+ "pos_z": 89.03125,
+ "stations": "Singer Landing,Haberlandt Point"
+ },
+ {
+ "name": "HIP 62772",
+ "pos_x": 8.3125,
+ "pos_y": 127.96875,
+ "pos_z": 7.125,
+ "stations": "King Platform,Littlewood Colony,Shaver's Claim"
+ },
+ {
+ "name": "HIP 62794",
+ "pos_x": 2.65625,
+ "pos_y": 122.4375,
+ "pos_z": 3.46875,
+ "stations": "Macarthur Terminal,Bardeen Installation,Russell Landing"
+ },
+ {
+ "name": "HIP 62809",
+ "pos_x": 130.9375,
+ "pos_y": 16.53125,
+ "pos_z": 86.46875,
+ "stations": "Shkaplerov Port,Usachov Settlement,Monge Penal colony"
+ },
+ {
+ "name": "HIP 62816",
+ "pos_x": -48.25,
+ "pos_y": 160.40625,
+ "pos_z": -29.34375,
+ "stations": "Metz Point,Kuttner Stop"
+ },
+ {
+ "name": "HIP 62857",
+ "pos_x": 0.03125,
+ "pos_y": 172.0625,
+ "pos_z": 3.21875,
+ "stations": "Burton Horizons,Santarem Holdings,Bethke Depot"
+ },
+ {
+ "name": "HIP 62904",
+ "pos_x": 15.375,
+ "pos_y": 144.6875,
+ "pos_z": 13.21875,
+ "stations": "Lavrador Station,Dickson Refinery,George Port"
+ },
+ {
+ "name": "HIP 62921",
+ "pos_x": 79.875,
+ "pos_y": 111.5625,
+ "pos_z": 55.375,
+ "stations": "Porsche Dock,Crown Platform"
+ },
+ {
+ "name": "HIP 62925",
+ "pos_x": 109.40625,
+ "pos_y": 93.34375,
+ "pos_z": 74.5625,
+ "stations": "Higginbotham Landing,Moseley Orbital"
+ },
+ {
+ "name": "HIP 63048",
+ "pos_x": -2.59375,
+ "pos_y": 139.375,
+ "pos_z": 2.25,
+ "stations": "Kovalevskaya Gateway,Shaikh Orbital,Hoffman Station,Ron Hubbard Ring,Polya Gateway,Slusser Station,Jenkinson Ring,Remek Installation,Fraser Beacon,Dowling Base"
+ },
+ {
+ "name": "HIP 63079",
+ "pos_x": 42.34375,
+ "pos_y": 189.6875,
+ "pos_z": 34,
+ "stations": "Cremona Dock,Litke Ring,Compton Bastion"
+ },
+ {
+ "name": "HIP 63181",
+ "pos_x": -20.15625,
+ "pos_y": 130.09375,
+ "pos_z": -8.90625,
+ "stations": "Vlamingh City,Sucharitkul Port"
+ },
+ {
+ "name": "HIP 63205",
+ "pos_x": 139.96875,
+ "pos_y": 7.1875,
+ "pos_z": 94.375,
+ "stations": "Wandrei Installation"
+ },
+ {
+ "name": "HIP 63207",
+ "pos_x": 122.46875,
+ "pos_y": 54.28125,
+ "pos_z": 84.375,
+ "stations": "Gessi Port,Regiomontanus Arena"
+ },
+ {
+ "name": "HIP 63235",
+ "pos_x": 61.09375,
+ "pos_y": 158.21875,
+ "pos_z": 47.40625,
+ "stations": "Robinson Base,Wilhelm Installation"
+ },
+ {
+ "name": "HIP 6324",
+ "pos_x": -74.625,
+ "pos_y": -116.59375,
+ "pos_z": -73.875,
+ "stations": "Van Scyoc Terminal,Crown Dock,Virtanen Works,Garden City"
+ },
+ {
+ "name": "HIP 63317",
+ "pos_x": -25.4375,
+ "pos_y": 141.5,
+ "pos_z": -10.90625,
+ "stations": "McMullen Prospect,Robinson Settlement"
+ },
+ {
+ "name": "HIP 63322",
+ "pos_x": -23.375,
+ "pos_y": 129.84375,
+ "pos_z": -10,
+ "stations": "Crowley Dock,Nourse Dock,Narita Installation,Forbes Dock,Stevin Relay"
+ },
+ {
+ "name": "HIP 63354",
+ "pos_x": 51.21875,
+ "pos_y": 160.875,
+ "pos_z": 42.1875,
+ "stations": "Rice Port"
+ },
+ {
+ "name": "HIP 63440",
+ "pos_x": -15.84375,
+ "pos_y": 172.9375,
+ "pos_z": -1.84375,
+ "stations": "Jones Arsenal,Piccard Outpost"
+ },
+ {
+ "name": "HIP 63506",
+ "pos_x": -31.84375,
+ "pos_y": 130.53125,
+ "pos_z": -14.5625,
+ "stations": "Grothendieck Freeport,Sheffield Landing"
+ },
+ {
+ "name": "HIP 63530",
+ "pos_x": 105.75,
+ "pos_y": 72.5,
+ "pos_z": 76.53125,
+ "stations": "Haber Relay,Crichton Landing"
+ },
+ {
+ "name": "HIP 63548",
+ "pos_x": 82.28125,
+ "pos_y": 144.3125,
+ "pos_z": 64.75,
+ "stations": "Van Scyoc Reach"
+ },
+ {
+ "name": "HIP 63581",
+ "pos_x": -12.03125,
+ "pos_y": 160.59375,
+ "pos_z": 1.34375,
+ "stations": "Wood Enterprise"
+ },
+ {
+ "name": "HIP 6369",
+ "pos_x": 151.15625,
+ "pos_y": -208.75,
+ "pos_z": 79.75,
+ "stations": "Phillips Landing,Paul Vision"
+ },
+ {
+ "name": "HIP 63708",
+ "pos_x": 129.75,
+ "pos_y": 104.4375,
+ "pos_z": 96.75,
+ "stations": "Mitchell Stop"
+ },
+ {
+ "name": "HIP 63809",
+ "pos_x": 102.96875,
+ "pos_y": 0.625,
+ "pos_z": 71.625,
+ "stations": "Acaba Stop,Sarafanov Base"
+ },
+ {
+ "name": "HIP 63831",
+ "pos_x": 103.875,
+ "pos_y": 86.40625,
+ "pos_z": 78.78125,
+ "stations": "Stanley Terminal,Silves' Inheritance,Clair Relay"
+ },
+ {
+ "name": "HIP 63862",
+ "pos_x": 127.21875,
+ "pos_y": 32.28125,
+ "pos_z": 91.15625,
+ "stations": "Anthony de la Roche Arsenal,Fraas Hub"
+ },
+ {
+ "name": "HIP 64006",
+ "pos_x": 115.03125,
+ "pos_y": 77.875,
+ "pos_z": 87.375,
+ "stations": "Pordenone Base,Webb Beacon,Thompson Vision"
+ },
+ {
+ "name": "HIP 64059",
+ "pos_x": 0.9375,
+ "pos_y": 121,
+ "pos_z": 11.375,
+ "stations": "Wul Port,Rocklynne Hub,Carlisle Terminal,Peral Point,Crown Landing"
+ },
+ {
+ "name": "HIP 6407",
+ "pos_x": -64.9375,
+ "pos_y": -158.28125,
+ "pos_z": -74.3125,
+ "stations": "Timofeyevich Enterprise,Landis Orbital,Cartan Dock,Eanes' Inheritance,Caidin Enterprise"
+ },
+ {
+ "name": "HIP 64076",
+ "pos_x": -81.34375,
+ "pos_y": 129.71875,
+ "pos_z": -45.71875,
+ "stations": "Gibson Terminal,Holub Settlement,Chwedyk Penal colony"
+ },
+ {
+ "name": "HIP 641",
+ "pos_x": -140.96875,
+ "pos_y": -18.21875,
+ "pos_z": -72.375,
+ "stations": "Geston Survey,Blackman Colony"
+ },
+ {
+ "name": "HIP 64128",
+ "pos_x": -65.1875,
+ "pos_y": 164.0625,
+ "pos_z": -30.8125,
+ "stations": "Rasmussen Ring,Maclaurin Hub,Robinson Terminal,Ayerdhal's Progress"
+ },
+ {
+ "name": "HIP 64131",
+ "pos_x": -10.71875,
+ "pos_y": 150.59375,
+ "pos_z": 6.46875,
+ "stations": "Caselberg Orbital,Stjepan Seljan Keep,Lie Survey"
+ },
+ {
+ "name": "HIP 6418",
+ "pos_x": 72.03125,
+ "pos_y": -233.5,
+ "pos_z": 16.0625,
+ "stations": "Baillaud Hub,Sarmiento de Gamboa Penal colony"
+ },
+ {
+ "name": "HIP 64203",
+ "pos_x": 73.34375,
+ "pos_y": 128.125,
+ "pos_z": 64.53125,
+ "stations": "Julian Terminal,Ewald Settlement,Descartes Base,Cabana Enterprise"
+ },
+ {
+ "name": "HIP 64208",
+ "pos_x": -78.8125,
+ "pos_y": 155.71875,
+ "pos_z": -40.71875,
+ "stations": "Gold Landing"
+ },
+ {
+ "name": "HIP 6422",
+ "pos_x": 90.78125,
+ "pos_y": -243.65625,
+ "pos_z": 28.4375,
+ "stations": "J. G. Ballard Base,Carroll Platform,Muramatsu Station,August von Steinheil Colony"
+ },
+ {
+ "name": "HIP 6439",
+ "pos_x": -82.53125,
+ "pos_y": -114.0625,
+ "pos_z": -80.71875,
+ "stations": "Pond Enterprise,Gilliland Enterprise"
+ },
+ {
+ "name": "HIP 6442",
+ "pos_x": -77.59375,
+ "pos_y": -140.59375,
+ "pos_z": -81.34375,
+ "stations": "Copernicus Landing,Grassmann Vision,Lawson Colony"
+ },
+ {
+ "name": "HIP 64443",
+ "pos_x": 133,
+ "pos_y": 88.75,
+ "pos_z": 105.59375,
+ "stations": "Shelley Asylum,Alexander Asylum"
+ },
+ {
+ "name": "HIP 64498",
+ "pos_x": 64.96875,
+ "pos_y": 155.8125,
+ "pos_z": 64.9375,
+ "stations": "Crook Gateway,Burstein's Pride"
+ },
+ {
+ "name": "HIP 64573",
+ "pos_x": 80.34375,
+ "pos_y": 109.84375,
+ "pos_z": 71.40625,
+ "stations": "Vries' Claim,Campbell Settlement,Drake's Inheritance"
+ },
+ {
+ "name": "HIP 64600",
+ "pos_x": -61.8125,
+ "pos_y": 110.90625,
+ "pos_z": -31.28125,
+ "stations": "Herbert Orbital,Barbosa Camp"
+ },
+ {
+ "name": "HIP 64626",
+ "pos_x": -75.3125,
+ "pos_y": 145.25,
+ "pos_z": -36.71875,
+ "stations": "Obruchev City,Hahn Orbital,Sternberg Gateway,Dorsey Vision"
+ },
+ {
+ "name": "HIP 64698",
+ "pos_x": 35.21875,
+ "pos_y": 166.4375,
+ "pos_z": 46.9375,
+ "stations": "Heceta Station,Nolan Dock,Paulo da Gama Base,Hill Keep"
+ },
+ {
+ "name": "HIP 64706",
+ "pos_x": 24.8125,
+ "pos_y": 148.875,
+ "pos_z": 37.1875,
+ "stations": "Clough Survey"
+ },
+ {
+ "name": "HIP 64714",
+ "pos_x": -68.53125,
+ "pos_y": 181.4375,
+ "pos_z": -26.59375,
+ "stations": "Hawkes Enterprise,Weyl Arsenal,Anthony de la Roche Dock,Isherwood Vision"
+ },
+ {
+ "name": "HIP 64726",
+ "pos_x": 42.59375,
+ "pos_y": 151.28125,
+ "pos_z": 50.71875,
+ "stations": "Moore Settlement,Drzewiecki Beacon,Elcano Installation"
+ },
+ {
+ "name": "HIP 64761",
+ "pos_x": -8.4375,
+ "pos_y": 129.5625,
+ "pos_z": 10.875,
+ "stations": "Sutcliffe Settlement"
+ },
+ {
+ "name": "HIP 64783",
+ "pos_x": 62.5,
+ "pos_y": 134,
+ "pos_z": 63.59375,
+ "stations": "Tucker Platform,Blair Freeport,Speke Vision"
+ },
+ {
+ "name": "HIP 64794",
+ "pos_x": 26.53125,
+ "pos_y": 170.09375,
+ "pos_z": 42.1875,
+ "stations": "von Bellingshausen Escape"
+ },
+ {
+ "name": "HIP 64848",
+ "pos_x": 0.65625,
+ "pos_y": 154.4375,
+ "pos_z": 21.75,
+ "stations": "McKay Base,Archer Dock"
+ },
+ {
+ "name": "HIP 64861",
+ "pos_x": -104.25,
+ "pos_y": 78.90625,
+ "pos_z": -65.71875,
+ "stations": "Chamitoff Enterprise,Fairbairn Enterprise,Stephenson City,Readdy Ring,Bowen City,Al-Farabi Orbital"
+ },
+ {
+ "name": "HIP 64872",
+ "pos_x": -39.625,
+ "pos_y": 120.0625,
+ "pos_z": -12.4375,
+ "stations": "Reis Depot"
+ },
+ {
+ "name": "HIP 64905",
+ "pos_x": 93.9375,
+ "pos_y": 140.9375,
+ "pos_z": 89.09375,
+ "stations": "Janszoon Bastion"
+ },
+ {
+ "name": "HIP 64934",
+ "pos_x": 97.15625,
+ "pos_y": 10.4375,
+ "pos_z": 73.1875,
+ "stations": "Coleman Mine,Molina Settlement,Lawhead's Progress"
+ },
+ {
+ "name": "HIP 64957",
+ "pos_x": 146.53125,
+ "pos_y": 29.625,
+ "pos_z": 112.53125,
+ "stations": "Clifford Works"
+ },
+ {
+ "name": "HIP 65036",
+ "pos_x": 116.65625,
+ "pos_y": -21.21875,
+ "pos_z": 83.375,
+ "stations": "Hodgson Vision,Somerville Settlement"
+ },
+ {
+ "name": "HIP 65049",
+ "pos_x": -34.0625,
+ "pos_y": 153.03125,
+ "pos_z": -2.28125,
+ "stations": "Serre Vision,Balmer Prospect,Gaiman Vision,Stafford Depot"
+ },
+ {
+ "name": "HIP 6510",
+ "pos_x": -34,
+ "pos_y": -207.125,
+ "pos_z": -60.59375,
+ "stations": "Thesiger Camp"
+ },
+ {
+ "name": "HIP 65114",
+ "pos_x": -39.1875,
+ "pos_y": 193.625,
+ "pos_z": 0.5625,
+ "stations": "Tsunenaga Platform,Alten Silo"
+ },
+ {
+ "name": "HIP 6512",
+ "pos_x": -123.21875,
+ "pos_y": -86.25,
+ "pos_z": -107.4375,
+ "stations": "Steele Mines,Taylor Point,Tennyson d'Eyncourt Depot"
+ },
+ {
+ "name": "HIP 65135",
+ "pos_x": -46.46875,
+ "pos_y": 149.21875,
+ "pos_z": -11.5625,
+ "stations": "Taylor Orbital,Stabenow Prospect,Poncelet Keep"
+ },
+ {
+ "name": "HIP 65165",
+ "pos_x": -60.78125,
+ "pos_y": 99.8125,
+ "pos_z": -29.71875,
+ "stations": "Madsen Colony,Huberath Reach"
+ },
+ {
+ "name": "HIP 65216",
+ "pos_x": -0.28125,
+ "pos_y": 182.78125,
+ "pos_z": 29.0625,
+ "stations": "Stairs Horizons,Strzelecki Installation"
+ },
+ {
+ "name": "HIP 65268",
+ "pos_x": -10.0625,
+ "pos_y": 176.84375,
+ "pos_z": 21.34375,
+ "stations": "Taylor Enterprise,Lopez de Legazpi Terminal,Regiomontanus Hub"
+ },
+ {
+ "name": "HIP 65276",
+ "pos_x": -59.15625,
+ "pos_y": 162,
+ "pos_z": -17.8125,
+ "stations": "Webb Hub,Russell Survey,Harris Orbital,Pohl Platform"
+ },
+ {
+ "name": "HIP 65346",
+ "pos_x": 131.34375,
+ "pos_y": 78.84375,
+ "pos_z": 112.28125,
+ "stations": "May Hub"
+ },
+ {
+ "name": "HIP 65371",
+ "pos_x": 19.875,
+ "pos_y": 127.65625,
+ "pos_z": 36.71875,
+ "stations": "Wagner City"
+ },
+ {
+ "name": "HIP 65429",
+ "pos_x": -103.875,
+ "pos_y": 82.375,
+ "pos_z": -64.28125,
+ "stations": "Clerk Survey"
+ },
+ {
+ "name": "HIP 65469",
+ "pos_x": -30.96875,
+ "pos_y": 126.4375,
+ "pos_z": -1.15625,
+ "stations": "Lasswitz Orbital,Hennepin Point"
+ },
+ {
+ "name": "HIP 65470",
+ "pos_x": 173.53125,
+ "pos_y": -62.53125,
+ "pos_z": 120.59375,
+ "stations": "Tavernier Horizons,Cady Barracks"
+ },
+ {
+ "name": "HIP 65485",
+ "pos_x": -45.59375,
+ "pos_y": 151.40625,
+ "pos_z": -7.75,
+ "stations": "Crown Dock,Judson Penal colony"
+ },
+ {
+ "name": "HIP 6551",
+ "pos_x": 22.09375,
+ "pos_y": -157.09375,
+ "pos_z": -10.28125,
+ "stations": "Schoening Enterprise,Pinto Base,Hagihara Prospect"
+ },
+ {
+ "name": "HIP 65557",
+ "pos_x": 120.6875,
+ "pos_y": 85.875,
+ "pos_z": 107.8125,
+ "stations": "Thirsk Depot"
+ },
+ {
+ "name": "HIP 65626",
+ "pos_x": 102.4375,
+ "pos_y": 54.90625,
+ "pos_z": 88.75,
+ "stations": "Burnell Arsenal,Blenkinsop Mines"
+ },
+ {
+ "name": "HIP 65632",
+ "pos_x": 16.9375,
+ "pos_y": 126.125,
+ "pos_z": 36.75,
+ "stations": "Springer Dock,Gordon Platform,Coney Depot,Northrop Hub"
+ },
+ {
+ "name": "HIP 65636",
+ "pos_x": 69.6875,
+ "pos_y": 82.5,
+ "pos_z": 68.96875,
+ "stations": "Rusch Orbital,Cavalieri Enterprise,Bokeili Port,MacLeod Station,Freycinet Dock,Hyecho Orbital,Pinzon Dock,Maler Orbital,Dyr Dock,Erikson Colony,Kettle's Progress,Zindell's Pride,RenenBellot Barracks"
+ },
+ {
+ "name": "HIP 65708",
+ "pos_x": 42.84375,
+ "pos_y": 124.75,
+ "pos_z": 57.0625,
+ "stations": "Leinster Plant,Stein Relay"
+ },
+ {
+ "name": "HIP 65758",
+ "pos_x": 148.21875,
+ "pos_y": -78.96875,
+ "pos_z": 98.75,
+ "stations": "Gehrels Base,Medupe Vision,Myasishchev Prospect"
+ },
+ {
+ "name": "HIP 65775",
+ "pos_x": -35.875,
+ "pos_y": 118.53125,
+ "pos_z": -4.28125,
+ "stations": "O'Leary Survey"
+ },
+ {
+ "name": "HIP 65780",
+ "pos_x": 24.875,
+ "pos_y": 179.40625,
+ "pos_z": 54.625,
+ "stations": "Roberts Camp"
+ },
+ {
+ "name": "HIP 65900",
+ "pos_x": -62.78125,
+ "pos_y": 184.125,
+ "pos_z": -10.96875,
+ "stations": "Merchiston Horizons,Houtman Orbital,Holland Dock,Harris Beacon"
+ },
+ {
+ "name": "HIP 65921",
+ "pos_x": -9.875,
+ "pos_y": 195.15625,
+ "pos_z": 32.71875,
+ "stations": "Mawson Port,Giles Laboratory"
+ },
+ {
+ "name": "HIP 65956",
+ "pos_x": 73.0625,
+ "pos_y": 101.09375,
+ "pos_z": 78.09375,
+ "stations": "Morey Park,Koch Orbital,Pontes City"
+ },
+ {
+ "name": "HIP 6596",
+ "pos_x": -141.34375,
+ "pos_y": 6.96875,
+ "pos_z": -105.8125,
+ "stations": "Artin Hub,Lupoff Outpost"
+ },
+ {
+ "name": "HIP 65963",
+ "pos_x": 59.78125,
+ "pos_y": 125.84375,
+ "pos_z": 73.03125,
+ "stations": "Brahe Legacy,Ellis Arena"
+ },
+ {
+ "name": "HIP 65971",
+ "pos_x": -72.5,
+ "pos_y": 123.8125,
+ "pos_z": -30.4375,
+ "stations": "Steinmuller Dock,Goeschke Plant"
+ },
+ {
+ "name": "HIP 66262",
+ "pos_x": -51.78125,
+ "pos_y": 131.5625,
+ "pos_z": -10.875,
+ "stations": "Whymper Port,Murphy Ring,Alexandria Enterprise,Pook Point"
+ },
+ {
+ "name": "HIP 66267",
+ "pos_x": -100.34375,
+ "pos_y": 105.0625,
+ "pos_z": -55.3125,
+ "stations": "Eisenstein Port,Bering Port"
+ },
+ {
+ "name": "HIP 66290",
+ "pos_x": -5.5,
+ "pos_y": 147.9375,
+ "pos_z": 29.71875,
+ "stations": "Smith Dock,Dashiell Settlement,Egan Prospect"
+ },
+ {
+ "name": "HIP 66322",
+ "pos_x": 84.0625,
+ "pos_y": 100.875,
+ "pos_z": 90.0625,
+ "stations": "Avdeyev Holdings,Bryant Bastion"
+ },
+ {
+ "name": "HIP 66514",
+ "pos_x": -40.1875,
+ "pos_y": 144.5,
+ "pos_z": 3.34375,
+ "stations": "Piercy's Progress"
+ },
+ {
+ "name": "HIP 66530",
+ "pos_x": 85.34375,
+ "pos_y": 96.65625,
+ "pos_z": 92.34375,
+ "stations": "Chamitoff Hangar,Titov Outpost"
+ },
+ {
+ "name": "HIP 66547",
+ "pos_x": 113.71875,
+ "pos_y": 67.4375,
+ "pos_z": 108.0625,
+ "stations": "Kratman Stop"
+ },
+ {
+ "name": "HIP 66730",
+ "pos_x": -0.90625,
+ "pos_y": 184.5,
+ "pos_z": 47.375,
+ "stations": "Yourkevitch Colony"
+ },
+ {
+ "name": "HIP 66754",
+ "pos_x": 113.4375,
+ "pos_y": 18.375,
+ "pos_z": 96.84375,
+ "stations": "Jendrassik Settlement,Babbage Platform"
+ },
+ {
+ "name": "HIP 66814",
+ "pos_x": 105.65625,
+ "pos_y": -0.5,
+ "pos_z": 85.875,
+ "stations": "Song Platform,Acton Horizons,Kuttner Silo"
+ },
+ {
+ "name": "HIP 66828",
+ "pos_x": -5.15625,
+ "pos_y": 129.25,
+ "pos_z": 30.28125,
+ "stations": "Auer Holdings,Appel Base"
+ },
+ {
+ "name": "HIP 66854",
+ "pos_x": 103.875,
+ "pos_y": 32.78125,
+ "pos_z": 93.5,
+ "stations": "Hasse Laboratory,Chorel's Folly"
+ },
+ {
+ "name": "HIP 66901",
+ "pos_x": 68.21875,
+ "pos_y": 127.75,
+ "pos_z": 90.4375,
+ "stations": "Avogadro Colony,Ferguson Orbital,Grothendieck Landing"
+ },
+ {
+ "name": "HIP 66974",
+ "pos_x": 9.3125,
+ "pos_y": 164,
+ "pos_z": 53.125,
+ "stations": "Piccard Dock"
+ },
+ {
+ "name": "HIP 66987",
+ "pos_x": 49.1875,
+ "pos_y": 104.3125,
+ "pos_z": 69.375,
+ "stations": "Feynman Terminal"
+ },
+ {
+ "name": "HIP 67009",
+ "pos_x": 128.15625,
+ "pos_y": 65.0625,
+ "pos_z": 123.5,
+ "stations": "Person Dock,Fanning Enterprise,Shumil Settlement"
+ },
+ {
+ "name": "HIP 6703",
+ "pos_x": 119.15625,
+ "pos_y": -155.71875,
+ "pos_z": 62.4375,
+ "stations": "Marsden Port,Regiomontanus City"
+ },
+ {
+ "name": "HIP 67031",
+ "pos_x": 44.90625,
+ "pos_y": 100.09375,
+ "pos_z": 65.03125,
+ "stations": "Mayr Station"
+ },
+ {
+ "name": "HIP 67055",
+ "pos_x": 63.9375,
+ "pos_y": 98.375,
+ "pos_z": 80.46875,
+ "stations": "Fettman Gateway,Garratt Gateway,Ivins Terminal,Simak Base,Zholobov Enterprise,Tani Hub,Wiley Gateway,Rushd Ring"
+ },
+ {
+ "name": "HIP 67058",
+ "pos_x": -78.4375,
+ "pos_y": 96.8125,
+ "pos_z": -37.28125,
+ "stations": "Dietz Vision,Vonarburg Terminal,Niven Hub,Wilder Station,Kepler Depot"
+ },
+ {
+ "name": "HIP 67086",
+ "pos_x": 38.96875,
+ "pos_y": 158.9375,
+ "pos_z": 77.53125,
+ "stations": "Allen Survey,Volynov Base"
+ },
+ {
+ "name": "HIP 67109",
+ "pos_x": -88.8125,
+ "pos_y": 107.71875,
+ "pos_z": -42.5,
+ "stations": "Hermite's Progress,Gallun Refinery,Napier Reach"
+ },
+ {
+ "name": "HIP 6712",
+ "pos_x": -39.90625,
+ "pos_y": -157.34375,
+ "pos_z": -59.21875,
+ "stations": "Mastracchio Installation"
+ },
+ {
+ "name": "HIP 67152",
+ "pos_x": 30.15625,
+ "pos_y": 118.625,
+ "pos_z": 59.25,
+ "stations": "Goddard Vision,Priestley Terminal,Kubasov Gateway,Ampere Horizons,Phillips Prospect"
+ },
+ {
+ "name": "HIP 67205",
+ "pos_x": 123.25,
+ "pos_y": -16.90625,
+ "pos_z": 97.40625,
+ "stations": "Braun Mines"
+ },
+ {
+ "name": "HIP 67389",
+ "pos_x": 92.03125,
+ "pos_y": 58.875,
+ "pos_z": 95.25,
+ "stations": "Cook Terminal,Barentsz Station,Needham Landing"
+ },
+ {
+ "name": "HIP 67412",
+ "pos_x": 31.0625,
+ "pos_y": 103.9375,
+ "pos_z": 58.125,
+ "stations": "Fullerton Horizons,Napier Horizons,Emshwiller Settlement"
+ },
+ {
+ "name": "HIP 67438",
+ "pos_x": 123.09375,
+ "pos_y": 65.84375,
+ "pos_z": 123.9375,
+ "stations": "Bujold Dock,MacVicar's Inheritance,Elwood Depot,VanderMeer Park"
+ },
+ {
+ "name": "HIP 67458",
+ "pos_x": 72.15625,
+ "pos_y": 75.75,
+ "pos_z": 84.3125,
+ "stations": "Culpeper Legacy,Lawson Camp"
+ },
+ {
+ "name": "HIP 67470",
+ "pos_x": 11,
+ "pos_y": 182.625,
+ "pos_z": 66.25,
+ "stations": "Bentham Relay,Daniel Vision"
+ },
+ {
+ "name": "HIP 67527",
+ "pos_x": 132.46875,
+ "pos_y": 54.46875,
+ "pos_z": 128.96875,
+ "stations": "Diamond Vision,Griffith Works,Swift Dock,Conway Terminal"
+ },
+ {
+ "name": "HIP 6758",
+ "pos_x": 30.28125,
+ "pos_y": -223.875,
+ "pos_z": -18.5625,
+ "stations": "Esclangon Platform,Burnham Station"
+ },
+ {
+ "name": "HIP 6761",
+ "pos_x": 35.6875,
+ "pos_y": -263.625,
+ "pos_z": -21.84375,
+ "stations": "Mori Vision,Kidinnu Keep"
+ },
+ {
+ "name": "HIP 6772",
+ "pos_x": 80.1875,
+ "pos_y": -177.5625,
+ "pos_z": 28.15625,
+ "stations": "Banneker Hub,Haro Orbital"
+ },
+ {
+ "name": "HIP 6780",
+ "pos_x": 135.96875,
+ "pos_y": -256.5625,
+ "pos_z": 55.96875,
+ "stations": "Adams Works,Watts Base"
+ },
+ {
+ "name": "HIP 67863",
+ "pos_x": 134.84375,
+ "pos_y": 49.25,
+ "pos_z": 132.375,
+ "stations": "Rolland Depot"
+ },
+ {
+ "name": "HIP 67882",
+ "pos_x": 13.0625,
+ "pos_y": 147.21875,
+ "pos_z": 61.125,
+ "stations": "Adams Station,Ryumin Settlement,Porsche Terminal,Clayton Horizons"
+ },
+ {
+ "name": "HIP 67918",
+ "pos_x": 109.40625,
+ "pos_y": 80.0625,
+ "pos_z": 121.53125,
+ "stations": "Slusser Camp"
+ },
+ {
+ "name": "HIP 67921",
+ "pos_x": -46.84375,
+ "pos_y": 162.21875,
+ "pos_z": 15.15625,
+ "stations": "Nagata Installation,Liska Hangar,Ali Hub"
+ },
+ {
+ "name": "HIP 67934",
+ "pos_x": 74.84375,
+ "pos_y": 102.5,
+ "pos_z": 99.53125,
+ "stations": "Fontenay Prospect"
+ },
+ {
+ "name": "HIP 6795",
+ "pos_x": 9.34375,
+ "pos_y": -141.59375,
+ "pos_z": -19.59375,
+ "stations": "Schiaparelli Station,Gotz Platform,Lyot Plant,Sweet Terminal"
+ },
+ {
+ "name": "HIP 6796",
+ "pos_x": -69.09375,
+ "pos_y": -76.84375,
+ "pos_z": -67.4375,
+ "stations": "Ibold Port,Kirtley Vision,Nylund Arsenal,al-Khowarizmi Orbital,Navigator Station"
+ },
+ {
+ "name": "HIP 67967",
+ "pos_x": 51.96875,
+ "pos_y": 123.875,
+ "pos_z": 87.53125,
+ "stations": "Stephenson Port"
+ },
+ {
+ "name": "HIP 6807",
+ "pos_x": 10.59375,
+ "pos_y": -202.375,
+ "pos_z": -30.25,
+ "stations": "Eisinga Colony,Young Orbital,Amano Dock,Herreshoff Barracks"
+ },
+ {
+ "name": "HIP 68085",
+ "pos_x": -105.84375,
+ "pos_y": 138.90625,
+ "pos_z": -42.8125,
+ "stations": "Verne Orbital,Hoyle Works,Escobar Enterprise"
+ },
+ {
+ "name": "HIP 68107",
+ "pos_x": 47.78125,
+ "pos_y": 107.15625,
+ "pos_z": 79.40625,
+ "stations": "Teng Installation,Potrykus Hangar,Al-Farabi Installation"
+ },
+ {
+ "name": "HIP 68158",
+ "pos_x": 7.4375,
+ "pos_y": 132.875,
+ "pos_z": 53.96875,
+ "stations": "Wandrei Settlement,Reynolds' Progress,Lee Orbital"
+ },
+ {
+ "name": "HIP 68160",
+ "pos_x": -12.6875,
+ "pos_y": 117.84375,
+ "pos_z": 31.125,
+ "stations": "Burstein Enterprise,Waldeck Enterprise,Holberg Terminal,Ryman Orbital,Chaviano Hub,Gernsback City,Fernandes Hub"
+ },
+ {
+ "name": "HIP 68162",
+ "pos_x": 108.03125,
+ "pos_y": 13.5625,
+ "pos_z": 98.90625,
+ "stations": "Silverberg Vision,Forfait Orbital,Samos Plant"
+ },
+ {
+ "name": "HIP 68193",
+ "pos_x": -79.0625,
+ "pos_y": 152.53125,
+ "pos_z": -14,
+ "stations": "Amis Station,Abel Platform,Reynolds Depot"
+ },
+ {
+ "name": "HIP 68204",
+ "pos_x": -85.1875,
+ "pos_y": 145.65625,
+ "pos_z": -21.75,
+ "stations": "Bulgakov Plant,Delany Survey,Ahmed Platform"
+ },
+ {
+ "name": "HIP 68434",
+ "pos_x": 70.03125,
+ "pos_y": 76.25,
+ "pos_z": 90.71875,
+ "stations": "Kroehl Station,Serrao City,Sohl Dock,Drummond City,Archer Escape,Godwin Hub,Amnuel Bastion,Brosnan Dock,Tavares Installation,Qian Reach,Kelly Stop,Sturgeon Relay,Pinzon Point"
+ },
+ {
+ "name": "HIP 6847",
+ "pos_x": -9.90625,
+ "pos_y": -177.84375,
+ "pos_z": -41.78125,
+ "stations": "Albitzky Platform,Keyes Escape"
+ },
+ {
+ "name": "HIP 68522",
+ "pos_x": -46.9375,
+ "pos_y": 168.0625,
+ "pos_z": 23.09375,
+ "stations": "Lem Survey,Marlowe Colony"
+ },
+ {
+ "name": "HIP 6854",
+ "pos_x": 16.6875,
+ "pos_y": -171.78125,
+ "pos_z": -20.21875,
+ "stations": "Baille Vision,Korolyov Dock,Rizvi Port,Corte-Real Lab,Bates Survey,Haber Escape"
+ },
+ {
+ "name": "HIP 68547",
+ "pos_x": -15.3125,
+ "pos_y": 160,
+ "pos_z": 48.3125,
+ "stations": "Anthony Horizons,Clement Barracks"
+ },
+ {
+ "name": "HIP 68552",
+ "pos_x": 73.75,
+ "pos_y": 101.4375,
+ "pos_z": 104.84375,
+ "stations": "Tapinas Installation"
+ },
+ {
+ "name": "HIP 68593",
+ "pos_x": -28.875,
+ "pos_y": 122.84375,
+ "pos_z": 22.25,
+ "stations": "Arkwright Settlement,Fossum City,Abnett Vista"
+ },
+ {
+ "name": "HIP 68602",
+ "pos_x": 101.75,
+ "pos_y": 71.03125,
+ "pos_z": 118.4375,
+ "stations": "Ferguson Mine"
+ },
+ {
+ "name": "HIP 68688",
+ "pos_x": 91.3125,
+ "pos_y": 97,
+ "pos_z": 120.28125,
+ "stations": "Cixin Beacon"
+ },
+ {
+ "name": "HIP 68734",
+ "pos_x": -47.59375,
+ "pos_y": 149.6875,
+ "pos_z": 17.34375,
+ "stations": "Griffin Dock,Keyes Observatory"
+ },
+ {
+ "name": "HIP 68736",
+ "pos_x": 117.40625,
+ "pos_y": 58.8125,
+ "pos_z": 128.96875,
+ "stations": "Rodrigues Installation"
+ },
+ {
+ "name": "HIP 68783",
+ "pos_x": 18.03125,
+ "pos_y": 125.75,
+ "pos_z": 67.09375,
+ "stations": "Arnarson Hub,Clute Beacon"
+ },
+ {
+ "name": "HIP 68785",
+ "pos_x": -22.25,
+ "pos_y": 137.28125,
+ "pos_z": 35.5625,
+ "stations": "Collins Holdings,Selous' Inheritance"
+ },
+ {
+ "name": "HIP 68796",
+ "pos_x": -94.34375,
+ "pos_y": 94.1875,
+ "pos_z": -46.6875,
+ "stations": "Smith Colony,Pelt's Pride"
+ },
+ {
+ "name": "HIP 68801",
+ "pos_x": 8.5,
+ "pos_y": 140.0625,
+ "pos_z": 64.625,
+ "stations": "Huxley Prospect,Larbalestier Refinery,Napier Base"
+ },
+ {
+ "name": "HIP 68805",
+ "pos_x": 47.53125,
+ "pos_y": 119.125,
+ "pos_z": 91.375,
+ "stations": "Snodgrass Enterprise,Hill's Pride,Payson Settlement"
+ },
+ {
+ "name": "HIP 68900",
+ "pos_x": 49.84375,
+ "pos_y": 87.65625,
+ "pos_z": 81.59375,
+ "stations": "Nylund Beacon"
+ },
+ {
+ "name": "HIP 68936",
+ "pos_x": 34,
+ "pos_y": 105.78125,
+ "pos_z": 74.96875,
+ "stations": "Marshburn Orbital,Lee Legacy"
+ },
+ {
+ "name": "HIP 69012",
+ "pos_x": 104,
+ "pos_y": 28.375,
+ "pos_z": 106.8125,
+ "stations": "Musa al-Khwarizmi Orbital,Mitchell Platform,Hoyle Hub"
+ },
+ {
+ "name": "HIP 69028",
+ "pos_x": -12.125,
+ "pos_y": 133.59375,
+ "pos_z": 45.71875,
+ "stations": "Ackerman Sanctuary"
+ },
+ {
+ "name": "HIP 6905",
+ "pos_x": 33.28125,
+ "pos_y": -214.6875,
+ "pos_z": -16.5625,
+ "stations": "Yuzhe Dock,Harawi Terminal,Angstrom Hub,Hendel Installation,Ibold Penal colony"
+ },
+ {
+ "name": "HIP 69050",
+ "pos_x": -76.6875,
+ "pos_y": 172.53125,
+ "pos_z": 3.5,
+ "stations": "Harper Mines"
+ },
+ {
+ "name": "HIP 69074",
+ "pos_x": 127.40625,
+ "pos_y": 21.5625,
+ "pos_z": 125.6875,
+ "stations": "Lehtonen Dock,Ellison Lab,Polya Installation"
+ },
+ {
+ "name": "HIP 69124",
+ "pos_x": -68.03125,
+ "pos_y": 188.53125,
+ "pos_z": 19.15625,
+ "stations": "Herodotus Dock,Cavalieri Enterprise,Harper Penal colony"
+ },
+ {
+ "name": "HIP 69129",
+ "pos_x": 112.65625,
+ "pos_y": 24.625,
+ "pos_z": 113.90625,
+ "stations": "Burnet Orbital,Thuot Station"
+ },
+ {
+ "name": "HIP 6913",
+ "pos_x": -105.0625,
+ "pos_y": 57.125,
+ "pos_z": -69.78125,
+ "stations": "Blaha Dock,Parker Settlement,Haldeman Point"
+ },
+ {
+ "name": "HIP 6921",
+ "pos_x": 40.09375,
+ "pos_y": -223.5,
+ "pos_z": -13.25,
+ "stations": "Pilyugin Terminal,Artsutanov Vision,Paczynski Installation,LeConte's Pride,Blackman's Inheritance"
+ },
+ {
+ "name": "HIP 69230",
+ "pos_x": -30.4375,
+ "pos_y": 150.15625,
+ "pos_z": 38.28125,
+ "stations": "Kidman Platform"
+ },
+ {
+ "name": "HIP 69240",
+ "pos_x": -108.5625,
+ "pos_y": 113.34375,
+ "pos_z": -50.0625,
+ "stations": "Williams Holdings,Phillips Platform,Marlowe Installation"
+ },
+ {
+ "name": "HIP 69400",
+ "pos_x": -81.59375,
+ "pos_y": 96.84375,
+ "pos_z": -31.84375,
+ "stations": "Bulychev Mines,Great Hub,Pullman Colony,Kneale Survey"
+ },
+ {
+ "name": "HIP 6943",
+ "pos_x": -108.84375,
+ "pos_y": -51.5625,
+ "pos_z": -94.375,
+ "stations": "Gorbatko Outpost,Emshwiller's Inheritance,Polansky Point"
+ },
+ {
+ "name": "HIP 69442",
+ "pos_x": -64.6875,
+ "pos_y": 106.03125,
+ "pos_z": -11.6875,
+ "stations": "Fiennes Depot,Krylov Enterprise,Payne Hub"
+ },
+ {
+ "name": "HIP 69457",
+ "pos_x": 149.3125,
+ "pos_y": -45.75,
+ "pos_z": 118.40625,
+ "stations": "Youll Port"
+ },
+ {
+ "name": "HIP 6949",
+ "pos_x": 104.84375,
+ "pos_y": -233.71875,
+ "pos_z": 34.375,
+ "stations": "Libeskind Vision,Whitcomb Vision,Speke Survey"
+ },
+ {
+ "name": "HIP 69518",
+ "pos_x": -52.0625,
+ "pos_y": 110.4375,
+ "pos_z": 2.5625,
+ "stations": "Great Port,Abnett City,Wright Port,Banks Terminal,Charnas City,Friend Station,Bombelli Port,Flinders Enterprise,Milnor Palace"
+ },
+ {
+ "name": "HIP 69699",
+ "pos_x": -84.5,
+ "pos_y": 131.9375,
+ "pos_z": -16.65625,
+ "stations": "Starzl Colony,Linge Station,Silverberg Arsenal,Waldrop Platform"
+ },
+ {
+ "name": "HIP 69709",
+ "pos_x": -70.25,
+ "pos_y": 131.28125,
+ "pos_z": -3.4375,
+ "stations": "Midgeley Survey"
+ },
+ {
+ "name": "HIP 69738",
+ "pos_x": -112.09375,
+ "pos_y": 168.40625,
+ "pos_z": -24.96875,
+ "stations": "Pinto Dock"
+ },
+ {
+ "name": "HIP 6975",
+ "pos_x": -88.125,
+ "pos_y": -97.0625,
+ "pos_z": -87.75,
+ "stations": "Haipeng's Progress"
+ },
+ {
+ "name": "HIP 69767",
+ "pos_x": -95.46875,
+ "pos_y": 112.84375,
+ "pos_z": -35.9375,
+ "stations": "Stjepan Seljan Camp,Sellings Settlement,Jeury's Inheritance"
+ },
+ {
+ "name": "HIP 6978",
+ "pos_x": -82.03125,
+ "pos_y": -67.03125,
+ "pos_z": -77,
+ "stations": "Plucker Dock,Sterling Station"
+ },
+ {
+ "name": "HIP 69783",
+ "pos_x": 16.625,
+ "pos_y": 124.0625,
+ "pos_z": 76.125,
+ "stations": "Tennyson d'Eyncourt Survey,Barnes Base"
+ },
+ {
+ "name": "HIP 69787",
+ "pos_x": -52.15625,
+ "pos_y": 159.3125,
+ "pos_z": 27.96875,
+ "stations": "Rangarajan Terminal,Abnett Prospect"
+ },
+ {
+ "name": "HIP 69796",
+ "pos_x": 107.8125,
+ "pos_y": -13.1875,
+ "pos_z": 96.0625,
+ "stations": "Delbruck Hub,Anders Port,Tall Vision"
+ },
+ {
+ "name": "HIP 69799",
+ "pos_x": 112.625,
+ "pos_y": 35.28125,
+ "pos_z": 124.25,
+ "stations": "Foreman Settlement"
+ },
+ {
+ "name": "HIP 6990",
+ "pos_x": 4.1875,
+ "pos_y": -172.4375,
+ "pos_z": -31.65625,
+ "stations": "Banno Landing,Littrow Oasis,Skolem Vision"
+ },
+ {
+ "name": "HIP 69901",
+ "pos_x": 91.71875,
+ "pos_y": 34.5625,
+ "pos_z": 104.84375,
+ "stations": "Vian Works"
+ },
+ {
+ "name": "HIP 69913",
+ "pos_x": -39.4375,
+ "pos_y": 157.25,
+ "pos_z": 40.40625,
+ "stations": "Kondratyev Outpost,Patrick Terminal"
+ },
+ {
+ "name": "HIP 69982",
+ "pos_x": 94.71875,
+ "pos_y": -0.28125,
+ "pos_z": 90.75,
+ "stations": "Moorcock Enterprise,Rondon Hub,Charnas Gateway,Covington Forum"
+ },
+ {
+ "name": "HIP 69985",
+ "pos_x": -30.78125,
+ "pos_y": 167.125,
+ "pos_z": 54.375,
+ "stations": "Cobb Dock,Vespucci Settlement"
+ },
+ {
+ "name": "HIP 69999",
+ "pos_x": -30.9375,
+ "pos_y": 137.6875,
+ "pos_z": 39.625,
+ "stations": "Charnas Terminal,Macomb City"
+ },
+ {
+ "name": "HIP 70025",
+ "pos_x": 15.34375,
+ "pos_y": 139.25,
+ "pos_z": 85.1875,
+ "stations": "Resnick Platform,Champlain Settlement"
+ },
+ {
+ "name": "HIP 70049",
+ "pos_x": 41.1875,
+ "pos_y": 103.375,
+ "pos_z": 92.28125,
+ "stations": "Mille Enterprise,Lethem Orbital,Regiomontanus Holdings"
+ },
+ {
+ "name": "HIP 70098",
+ "pos_x": 111.34375,
+ "pos_y": -9.53125,
+ "pos_z": 102.65625,
+ "stations": "Ore Settlement,Nachtigal Beacon"
+ },
+ {
+ "name": "HIP 70142",
+ "pos_x": -46.96875,
+ "pos_y": 128.46875,
+ "pos_z": 20.78125,
+ "stations": "Bruce Terminal,Nearchus Settlement,Bellamy Depot,Ozanne Survey,Cook Horizons"
+ },
+ {
+ "name": "HIP 7018",
+ "pos_x": 14.25,
+ "pos_y": -119.09375,
+ "pos_z": -13.3125,
+ "stations": "Noli Relay,Smith Observatory,Roth Station"
+ },
+ {
+ "name": "HIP 70239",
+ "pos_x": 137.21875,
+ "pos_y": -25.59375,
+ "pos_z": 120.125,
+ "stations": "Fairey Dock,Olahus Terminal"
+ },
+ {
+ "name": "HIP 70253",
+ "pos_x": -72.40625,
+ "pos_y": 112.59375,
+ "pos_z": -11.375,
+ "stations": "Galindo Station,Nixon Gateway,Manning City,Gerrold Hub,Garden Enterprise,Sladek Terminal,Svavarsson Dock,Bunch Bastion,Teng Point,Crowley Station,Rowley Terminal"
+ },
+ {
+ "name": "HIP 70255",
+ "pos_x": -99.28125,
+ "pos_y": 120.84375,
+ "pos_z": -33.21875,
+ "stations": "MacLean Colony,Ham Outpost"
+ },
+ {
+ "name": "HIP 70269",
+ "pos_x": 30.46875,
+ "pos_y": 95.78125,
+ "pos_z": 80.125,
+ "stations": "Overmyer Landing,Smith Hangar,Roberts Installation"
+ },
+ {
+ "name": "HIP 7037",
+ "pos_x": -123.40625,
+ "pos_y": -32.34375,
+ "pos_z": -102.5625,
+ "stations": "Reamy Sanctuary"
+ },
+ {
+ "name": "HIP 7040",
+ "pos_x": 108.28125,
+ "pos_y": -181.3125,
+ "pos_z": 46.71875,
+ "stations": "Leckie Barracks,Babcock Colony,Ponce de Leon Enterprise"
+ },
+ {
+ "name": "HIP 70470",
+ "pos_x": -52.53125,
+ "pos_y": 122.28125,
+ "pos_z": 14.59375,
+ "stations": "McIntyre Prospect,Garcia Base"
+ },
+ {
+ "name": "HIP 70487",
+ "pos_x": -89.21875,
+ "pos_y": 163.625,
+ "pos_z": 1.09375,
+ "stations": "Clairaut Dock"
+ },
+ {
+ "name": "HIP 70525",
+ "pos_x": 93.03125,
+ "pos_y": 86.6875,
+ "pos_z": 139.4375,
+ "stations": "Moskowitz's Progress,Tokubei Observatory"
+ },
+ {
+ "name": "HIP 7060",
+ "pos_x": 107.34375,
+ "pos_y": -240.125,
+ "pos_z": 33.59375,
+ "stations": "Myasishchev Depot,Niijima Colony"
+ },
+ {
+ "name": "HIP 70677",
+ "pos_x": 45.21875,
+ "pos_y": 96.375,
+ "pos_z": 99.125,
+ "stations": "Banach Relay,Matheson Legacy,Moresby Beacon"
+ },
+ {
+ "name": "HIP 70681",
+ "pos_x": 54.6875,
+ "pos_y": 96.1875,
+ "pos_z": 108.53125,
+ "stations": "Morris Terminal,Hermite Platform"
+ },
+ {
+ "name": "HIP 70714",
+ "pos_x": 41.5,
+ "pos_y": 96.5,
+ "pos_z": 95.875,
+ "stations": "Wilmore Refinery,Shuttleworth Relay"
+ },
+ {
+ "name": "HIP 70756",
+ "pos_x": 39.15625,
+ "pos_y": 78.21875,
+ "pos_z": 83.5625,
+ "stations": "Moskowitz Enterprise,Pytheas Dock,Reynolds Terminal,McKay Orbital,Norman Port,Coulter Vision,Romanenko Exchange,Garcia Dock,Egan Orbital,Matthews Hub,Aguirre Hub"
+ },
+ {
+ "name": "HIP 7076",
+ "pos_x": -21.9375,
+ "pos_y": -131.8125,
+ "pos_z": -44.5625,
+ "stations": "Kaku Hub,Yamazaki Gateway,He Survey"
+ },
+ {
+ "name": "HIP 70792",
+ "pos_x": -73.75,
+ "pos_y": 152.1875,
+ "pos_z": 12.96875,
+ "stations": "North Point"
+ },
+ {
+ "name": "HIP 7080",
+ "pos_x": -25.78125,
+ "pos_y": -181.78125,
+ "pos_z": -58.0625,
+ "stations": "Coggia Vision"
+ },
+ {
+ "name": "HIP 7082",
+ "pos_x": 127.3125,
+ "pos_y": -179.125,
+ "pos_z": 61.6875,
+ "stations": "Foster Vision"
+ },
+ {
+ "name": "HIP 70973",
+ "pos_x": 25.28125,
+ "pos_y": 100.6875,
+ "pos_z": 84.9375,
+ "stations": "Evans Colony,Van Scyoc Survey,Gann Port"
+ },
+ {
+ "name": "HIP 71050",
+ "pos_x": 98.8125,
+ "pos_y": -9.28125,
+ "pos_z": 95,
+ "stations": "Leavitt Platform"
+ },
+ {
+ "name": "HIP 7106",
+ "pos_x": 117.65625,
+ "pos_y": -196.90625,
+ "pos_z": 50.21875,
+ "stations": "Fedden Dock,Godel Point"
+ },
+ {
+ "name": "HIP 71122",
+ "pos_x": 85.125,
+ "pos_y": 92.09375,
+ "pos_z": 142.34375,
+ "stations": "Weiss Orbital,Darboux Platform,Baffin Landing,Sylvester Enterprise,Wilson Landing"
+ },
+ {
+ "name": "HIP 71165",
+ "pos_x": -65.0625,
+ "pos_y": 121.84375,
+ "pos_z": 7.3125,
+ "stations": "Garcia Refinery"
+ },
+ {
+ "name": "HIP 71193",
+ "pos_x": -41.625,
+ "pos_y": 109.90625,
+ "pos_z": 24.25,
+ "stations": "Bond Dock"
+ },
+ {
+ "name": "HIP 71247",
+ "pos_x": -9.5625,
+ "pos_y": 139.4375,
+ "pos_z": 75.78125,
+ "stations": "Verrazzano Port,Lopez de Villalobos Station,Fremion Port"
+ },
+ {
+ "name": "HIP 71276",
+ "pos_x": 18.375,
+ "pos_y": 111.0625,
+ "pos_z": 87.53125,
+ "stations": "J. G. Ballard Orbital,Danvers Enterprise,Peano Terminal,Shatner Prospect"
+ },
+ {
+ "name": "HIP 7136",
+ "pos_x": 98.8125,
+ "pos_y": -225.4375,
+ "pos_z": 29.1875,
+ "stations": "Arp Terminal,Kanai Palace,Bogdanov Reach"
+ },
+ {
+ "name": "HIP 7142",
+ "pos_x": 127.6875,
+ "pos_y": -147.71875,
+ "pos_z": 68.25,
+ "stations": "Truly Dock,Axon Dock,Babcock Orbital,Islam Dock"
+ },
+ {
+ "name": "HIP 71440",
+ "pos_x": -89.40625,
+ "pos_y": 128.78125,
+ "pos_z": -11.5,
+ "stations": "Howard Mines,Burbank Colony,Harness Observatory"
+ },
+ {
+ "name": "HIP 71462",
+ "pos_x": 27.9375,
+ "pos_y": 138.59375,
+ "pos_z": 117.1875,
+ "stations": "Celebi Terminal,Bunch Terminal,Weber Station"
+ },
+ {
+ "name": "HIP 71466",
+ "pos_x": -86.5,
+ "pos_y": 177.5,
+ "pos_z": 22.65625,
+ "stations": "Rotsler Landing,Sheffield Base"
+ },
+ {
+ "name": "HIP 71515",
+ "pos_x": 39.53125,
+ "pos_y": 81.71875,
+ "pos_z": 93.6875,
+ "stations": "Velho Dock,Resnick Platform,Rotsler Depot,Bear Plant"
+ },
+ {
+ "name": "HIP 71516",
+ "pos_x": 81.59375,
+ "pos_y": 84.3125,
+ "pos_z": 139.375,
+ "stations": "Herjulfsson Installation"
+ },
+ {
+ "name": "HIP 71527",
+ "pos_x": 8.125,
+ "pos_y": 129.9375,
+ "pos_z": 91.96875,
+ "stations": "Helms Enterprise,Torricelli Dock,Bessemer's Progress,Papin Orbital,Cardano Settlement"
+ },
+ {
+ "name": "HIP 7154",
+ "pos_x": 105.65625,
+ "pos_y": -229.21875,
+ "pos_z": 33.5,
+ "stations": "Schommer Mines"
+ },
+ {
+ "name": "HIP 71572",
+ "pos_x": -13.90625,
+ "pos_y": 135.09375,
+ "pos_z": 72.71875,
+ "stations": "McClintock Landing,Borman's Claim,Sellers Laboratory"
+ },
+ {
+ "name": "HIP 71625",
+ "pos_x": 87,
+ "pos_y": 63.875,
+ "pos_z": 133.21875,
+ "stations": "Friesner Keep,Leavitt Port,Alexandria Enterprise"
+ },
+ {
+ "name": "HIP 7167",
+ "pos_x": 50.0625,
+ "pos_y": -210.78125,
+ "pos_z": -6.21875,
+ "stations": "Naubakht Dock,Dummer Vista,Foerster Ring,Hubble Terminal,Watts Point,Markov Vision"
+ },
+ {
+ "name": "HIP 71679",
+ "pos_x": -107.53125,
+ "pos_y": 122.5625,
+ "pos_z": -32.875,
+ "stations": "Kapp Station,Hudson Legacy"
+ },
+ {
+ "name": "HIP 71682",
+ "pos_x": 57.78125,
+ "pos_y": 69.09375,
+ "pos_z": 106.5,
+ "stations": "Bachman Orbital,Fernandes Landing,Bunch Settlement,Townshend Survey,Gessi Landing"
+ },
+ {
+ "name": "HIP 71726",
+ "pos_x": -52.75,
+ "pos_y": 158.8125,
+ "pos_z": 49.46875,
+ "stations": "Marlowe Freeport"
+ },
+ {
+ "name": "HIP 71737",
+ "pos_x": -50.78125,
+ "pos_y": 152.15625,
+ "pos_z": 47.3125,
+ "stations": "Andrews Ring,Kozlov Penal colony"
+ },
+ {
+ "name": "HIP 71782",
+ "pos_x": -106.03125,
+ "pos_y": 145.78125,
+ "pos_z": -15.125,
+ "stations": "Crown Port,Simak Platform"
+ },
+ {
+ "name": "HIP 71788",
+ "pos_x": 36.09375,
+ "pos_y": 121.8125,
+ "pos_z": 120,
+ "stations": "Waldeck's Inheritance"
+ },
+ {
+ "name": "HIP 71931",
+ "pos_x": -23,
+ "pos_y": 179.78125,
+ "pos_z": 98.25,
+ "stations": "Cauchy Horizons,Crown Point"
+ },
+ {
+ "name": "HIP 71938",
+ "pos_x": -23.96875,
+ "pos_y": 187.1875,
+ "pos_z": 102.40625,
+ "stations": "Moon Camp"
+ },
+ {
+ "name": "HIP 7199",
+ "pos_x": 68.8125,
+ "pos_y": -232.53125,
+ "pos_z": 3.40625,
+ "stations": "de Kamp Orbital,Kowal Enterprise,Lambert Terminal"
+ },
+ {
+ "name": "HIP 72043",
+ "pos_x": -22,
+ "pos_y": 118.5,
+ "pos_z": 58.78125,
+ "stations": "Kippax Ring,Farouk Terminal,Grothendieck City,Brunel Station,Dunn Works,McCaffrey Works,Eschbach Horizons,Ivens Installation,Borel Gateway,Fremont Relay,Brothers Gateway"
+ },
+ {
+ "name": "HIP 7211",
+ "pos_x": 79.65625,
+ "pos_y": -167.9375,
+ "pos_z": 25.875,
+ "stations": "Mikoyan Orbital,Urata Installation,Tyson Vision,Veron Station,Winthrop Terminal"
+ },
+ {
+ "name": "HIP 7221",
+ "pos_x": -124.1875,
+ "pos_y": -25.5625,
+ "pos_z": -103,
+ "stations": "Erikson Dock,Womack Penal colony"
+ },
+ {
+ "name": "HIP 72221",
+ "pos_x": -7.90625,
+ "pos_y": 118.4375,
+ "pos_z": 76,
+ "stations": "Leslie Terminal,Murphy Relay"
+ },
+ {
+ "name": "HIP 72223",
+ "pos_x": 3.03125,
+ "pos_y": 101.09375,
+ "pos_z": 75.5625,
+ "stations": "Maler City,Laumer Arsenal,Anvil Port"
+ },
+ {
+ "name": "HIP 72235",
+ "pos_x": 64.1875,
+ "pos_y": 52.75,
+ "pos_z": 107.8125,
+ "stations": "Currie Horizons,Thornton Hub,Robinson Enterprise"
+ },
+ {
+ "name": "HIP 72336",
+ "pos_x": -28.8125,
+ "pos_y": 140.28125,
+ "pos_z": 70.1875,
+ "stations": "Kovalyonok Installation"
+ },
+ {
+ "name": "HIP 72353",
+ "pos_x": 66.4375,
+ "pos_y": 77.09375,
+ "pos_z": 129.09375,
+ "stations": "Weierstrass Arsenal,Caidin Station,Doyle Dock"
+ },
+ {
+ "name": "HIP 72380",
+ "pos_x": 137.71875,
+ "pos_y": -26.125,
+ "pos_z": 132.46875,
+ "stations": "Goodricke Oasis"
+ },
+ {
+ "name": "HIP 72423",
+ "pos_x": 40.96875,
+ "pos_y": 86.75,
+ "pos_z": 108.90625,
+ "stations": "Lefschetz Dock,Quimper Dock,Yolen Dock,Silverberg Station,Bunch Port,Siodmak Bastion"
+ },
+ {
+ "name": "HIP 7245",
+ "pos_x": -103,
+ "pos_y": -91.375,
+ "pos_z": -101.03125,
+ "stations": "Hale Horizons"
+ },
+ {
+ "name": "HIP 72479",
+ "pos_x": -10.375,
+ "pos_y": 120.96875,
+ "pos_z": 78.125,
+ "stations": "Nikolayev Settlement,Drzewiecki Survey,Dutton Depot"
+ },
+ {
+ "name": "HIP 726",
+ "pos_x": 21.75,
+ "pos_y": -189.59375,
+ "pos_z": 45.34375,
+ "stations": "Fozard Installation,Skiff Dock,Foss Enterprise"
+ },
+ {
+ "name": "HIP 72600",
+ "pos_x": 70.40625,
+ "pos_y": 62.6875,
+ "pos_z": 125.75,
+ "stations": "Wnuk-Lipinski Enterprise,Sheffield Terminal"
+ },
+ {
+ "name": "HIP 72604",
+ "pos_x": -29.1875,
+ "pos_y": 108.71875,
+ "pos_z": 49.40625,
+ "stations": "Bischoff Colony,Tiedemann Terminal,Bixby Beacon"
+ },
+ {
+ "name": "HIP 72663",
+ "pos_x": 12.15625,
+ "pos_y": 104.34375,
+ "pos_z": 93.03125,
+ "stations": "Sinclair Ring,Heisenberg Base"
+ },
+ {
+ "name": "HIP 72677",
+ "pos_x": 48.53125,
+ "pos_y": 101.9375,
+ "pos_z": 132.0625,
+ "stations": "Fourneyron Camp,Zudov Beacon,McCoy Terminal,Humphreys Point"
+ },
+ {
+ "name": "HIP 72703",
+ "pos_x": 3.40625,
+ "pos_y": 97.3125,
+ "pos_z": 78.28125,
+ "stations": "Egan Camp,Hippalus Legacy"
+ },
+ {
+ "name": "HIP 72726",
+ "pos_x": -103.71875,
+ "pos_y": 101.65625,
+ "pos_z": -38.5,
+ "stations": "Bokeili Settlement,Plexico Landing"
+ },
+ {
+ "name": "HIP 72753",
+ "pos_x": -42.78125,
+ "pos_y": 128.59375,
+ "pos_z": 50.84375,
+ "stations": "du Fresne Depot"
+ },
+ {
+ "name": "HIP 7277",
+ "pos_x": -76.59375,
+ "pos_y": -143.84375,
+ "pos_z": -92.25,
+ "stations": "Tayler Beacon,Pelliot Vision,Jakes Colony"
+ },
+ {
+ "name": "HIP 72797",
+ "pos_x": 83.0625,
+ "pos_y": 69.3125,
+ "pos_z": 147.25,
+ "stations": "Clerk Plant,Treshchov Reformatory"
+ },
+ {
+ "name": "HIP 7296",
+ "pos_x": -21.15625,
+ "pos_y": -149.3125,
+ "pos_z": -50.09375,
+ "stations": "Minkowski's Pride,Alvarado Barracks"
+ },
+ {
+ "name": "HIP 7298",
+ "pos_x": 127.78125,
+ "pos_y": -193.8125,
+ "pos_z": 57.28125,
+ "stations": "Stebbins Platform,Hamuy Survey,Maclaurin Penal colony"
+ },
+ {
+ "name": "HIP 72986",
+ "pos_x": 70.75,
+ "pos_y": 35.53125,
+ "pos_z": 108.84375,
+ "stations": "Barentsz Horizons,Dias Silo,Serrao's Claim,Barton Beacon"
+ },
+ {
+ "name": "HIP 72998",
+ "pos_x": 26.0625,
+ "pos_y": 109.0625,
+ "pos_z": 116.5,
+ "stations": "Sacco Stop,Al-Battani Works"
+ },
+ {
+ "name": "HIP 7304",
+ "pos_x": 27.25,
+ "pos_y": -211.40625,
+ "pos_z": -25.90625,
+ "stations": "Celsius Station,Kojima Station,Jefferies Vision"
+ },
+ {
+ "name": "HIP 73075",
+ "pos_x": 127.15625,
+ "pos_y": -42.03125,
+ "pos_z": 112,
+ "stations": "Gabriel Reformatory,Miller Colony"
+ },
+ {
+ "name": "HIP 73128",
+ "pos_x": -23.9375,
+ "pos_y": 153.09375,
+ "pos_z": 96.625,
+ "stations": "Clough Reformatory"
+ },
+ {
+ "name": "HIP 73205",
+ "pos_x": 84.875,
+ "pos_y": 43.15625,
+ "pos_z": 133.5625,
+ "stations": "la Cosa Relay"
+ },
+ {
+ "name": "HIP 73244",
+ "pos_x": 121.0625,
+ "pos_y": -26.5,
+ "pos_z": 118.5,
+ "stations": "Hoffman Enterprise,Menezes Keep,Caillie Port,Steele Port"
+ },
+ {
+ "name": "HIP 73269",
+ "pos_x": 59.25,
+ "pos_y": 66.71875,
+ "pos_z": 124.0625,
+ "stations": "Pournelle Terminal"
+ },
+ {
+ "name": "HIP 73293",
+ "pos_x": 145.84375,
+ "pos_y": -51.71875,
+ "pos_z": 126.625,
+ "stations": "Koolhaas' Folly,Matthaus Olbers Station,Ziegler Dock,Shea Hub,Gehry Plant,Sucharitkul Installation"
+ },
+ {
+ "name": "HIP 73314",
+ "pos_x": -7.4375,
+ "pos_y": 112.15625,
+ "pos_z": 84.6875,
+ "stations": "Hume Port,Malaspina Port,Hahn Station,de Balboa Terminal,Wallin City,Hodgson Depot,Spassky's Progress"
+ },
+ {
+ "name": "HIP 73321",
+ "pos_x": -35.40625,
+ "pos_y": 158.5,
+ "pos_z": 90.75,
+ "stations": "Birkhoff Ring,Effinger Port,Scheerbart Vision,Bretnor Base"
+ },
+ {
+ "name": "HIP 73370",
+ "pos_x": 39.375,
+ "pos_y": 77.40625,
+ "pos_z": 111,
+ "stations": "Laumer Dock"
+ },
+ {
+ "name": "HIP 7338",
+ "pos_x": -96.5,
+ "pos_y": 33.21875,
+ "pos_z": -68.65625,
+ "stations": "Hayden Barracks,Kirchoff Terminal,Bardeen Camp"
+ },
+ {
+ "name": "HIP 73408",
+ "pos_x": 132.59375,
+ "pos_y": -42.1875,
+ "pos_z": 119.6875,
+ "stations": "Bennett Dock"
+ },
+ {
+ "name": "HIP 73428",
+ "pos_x": 37.5625,
+ "pos_y": 91.53125,
+ "pos_z": 121.53125,
+ "stations": "Herodotus Colony"
+ },
+ {
+ "name": "HIP 73449",
+ "pos_x": -10.6875,
+ "pos_y": 103.09375,
+ "pos_z": 75,
+ "stations": "McArthur Port,Gentle Landing,Bass Survey"
+ },
+ {
+ "name": "HIP 7357",
+ "pos_x": -34.09375,
+ "pos_y": -104.09375,
+ "pos_z": -50.71875,
+ "stations": "Pauling Beacon,Schwann Terminal,Volynov City"
+ },
+ {
+ "name": "HIP 73577",
+ "pos_x": -39.4375,
+ "pos_y": 149.15625,
+ "pos_z": 82.53125,
+ "stations": "Bachman Dock,Nehsi Station"
+ },
+ {
+ "name": "HIP 73623",
+ "pos_x": -88.9375,
+ "pos_y": 95.9375,
+ "pos_z": -21.9375,
+ "stations": "Reynolds Dock"
+ },
+ {
+ "name": "HIP 73650",
+ "pos_x": 76.46875,
+ "pos_y": 51.9375,
+ "pos_z": 136.625,
+ "stations": "Ford Mines,Caryanda's Folly"
+ },
+ {
+ "name": "HIP 73677",
+ "pos_x": -89.96875,
+ "pos_y": 148.78125,
+ "pos_z": 23.5625,
+ "stations": "Shalatula Horizons"
+ },
+ {
+ "name": "HIP 7370",
+ "pos_x": -101.375,
+ "pos_y": -76.46875,
+ "pos_z": -97.71875,
+ "stations": "Kube-McDowell Ring,Neff Station,Barnaby Settlement,Spinrad Station"
+ },
+ {
+ "name": "HIP 73765",
+ "pos_x": -72.40625,
+ "pos_y": 138.1875,
+ "pos_z": 36.28125,
+ "stations": "Kanwar Arsenal,Watson Settlement,Cayley Hangar"
+ },
+ {
+ "name": "HIP 73814",
+ "pos_x": 79.6875,
+ "pos_y": 40.59375,
+ "pos_z": 132.3125,
+ "stations": "d'Eyncourt Port,Gardner Landing"
+ },
+ {
+ "name": "HIP 73846",
+ "pos_x": 16.25,
+ "pos_y": 104.5,
+ "pos_z": 113.625,
+ "stations": "Lovelace Settlement,Wegener Dock"
+ },
+ {
+ "name": "HIP 7386",
+ "pos_x": 32.84375,
+ "pos_y": -170.78125,
+ "pos_z": -13.3125,
+ "stations": "Henry Depot,Grushin's Claim,Albitzky Relay"
+ },
+ {
+ "name": "HIP 7395",
+ "pos_x": 104.21875,
+ "pos_y": -258.59375,
+ "pos_z": 22.8125,
+ "stations": "Adragna Landing,Barnwell Survey"
+ },
+ {
+ "name": "HIP 7396",
+ "pos_x": 2.28125,
+ "pos_y": -119.3125,
+ "pos_z": -25.78125,
+ "stations": "Seares Ring,Kepler Vision,Bode Dock"
+ },
+ {
+ "name": "HIP 74067",
+ "pos_x": 13.28125,
+ "pos_y": 80.6875,
+ "pos_z": 91.21875,
+ "stations": "Ozanne Ring,Alvarez de Pineda Station,Ackerman Terminal,Dantec Port,Powell's Inheritance,Kippax Dock,Forfait Orbital,Payne City,Tokubei Point,Koontz Camp,Stephens Installation"
+ },
+ {
+ "name": "HIP 74161",
+ "pos_x": -70.71875,
+ "pos_y": 102.75,
+ "pos_z": 9.53125,
+ "stations": "Olivas Base,Bear Mines,Kneale Point"
+ },
+ {
+ "name": "HIP 74174",
+ "pos_x": 43.9375,
+ "pos_y": 89.9375,
+ "pos_z": 138.75,
+ "stations": "Bradshaw Base,Maclaurin Horizons,Dampier Legacy"
+ },
+ {
+ "name": "HIP 74183",
+ "pos_x": -54.9375,
+ "pos_y": 151.59375,
+ "pos_z": 75.21875,
+ "stations": "Evans Station,Nicollier Settlement"
+ },
+ {
+ "name": "HIP 7420",
+ "pos_x": -95.5,
+ "pos_y": -46.21875,
+ "pos_z": -86.5,
+ "stations": "Morgan Terminal,Blackwell City,Kondakova Orbital,Northrop Oasis,Narita Bastion"
+ },
+ {
+ "name": "HIP 74243",
+ "pos_x": 70.59375,
+ "pos_y": 14.1875,
+ "pos_z": 100.90625,
+ "stations": "Foreman Refinery,Bean City,Westerfeld Installation"
+ },
+ {
+ "name": "HIP 74255",
+ "pos_x": 69.4375,
+ "pos_y": 12.25,
+ "pos_z": 97.78125,
+ "stations": "Tsibliyev Station,Guest City,Sarmiento de Gamboa Point,Vela City,Thomas Hub,Malpighi City,Lorentz Port,Shukor City"
+ },
+ {
+ "name": "HIP 74286",
+ "pos_x": -76.5,
+ "pos_y": 179.25,
+ "pos_z": 76.8125,
+ "stations": "Fuchs Depot,Plucker Mine"
+ },
+ {
+ "name": "HIP 74290",
+ "pos_x": 254.34375,
+ "pos_y": -39.0625,
+ "pos_z": 278.59375,
+ "stations": "Pratchett's Disc"
+ },
+ {
+ "name": "HIP 74304",
+ "pos_x": 1.3125,
+ "pos_y": 113.90625,
+ "pos_z": 111.28125,
+ "stations": "Acton Landing,Herzfeld Station,Lucretius Station,Brunel Survey,Drummond Penal colony"
+ },
+ {
+ "name": "HIP 74374",
+ "pos_x": -31.09375,
+ "pos_y": 117.34375,
+ "pos_z": 75.03125,
+ "stations": "Oefelein Hub,Bosch Base"
+ },
+ {
+ "name": "HIP 74381",
+ "pos_x": -73.4375,
+ "pos_y": 139.25,
+ "pos_z": 43.46875,
+ "stations": "Tesla Enterprise,Khrenov's Inheritance"
+ },
+ {
+ "name": "HIP 74405",
+ "pos_x": 117.875,
+ "pos_y": -42.96875,
+ "pos_z": 105.6875,
+ "stations": "Lundmark Dock,Baily Park,Cuffey Orbital,Arisman Vision,Thollon Prospect"
+ },
+ {
+ "name": "HIP 7441",
+ "pos_x": -123.46875,
+ "pos_y": -14.875,
+ "pos_z": -101.53125,
+ "stations": "Mitchell Orbital,Ross Gateway,Linnehan Enterprise,Goulart Beacon"
+ },
+ {
+ "name": "HIP 74466",
+ "pos_x": -118.96875,
+ "pos_y": 87.40625,
+ "pos_z": -63.59375,
+ "stations": "Libby City,Wolf Settlement"
+ },
+ {
+ "name": "HIP 74734",
+ "pos_x": -31.75,
+ "pos_y": 111.125,
+ "pos_z": 72.96875,
+ "stations": "Arrhenius Station,Polyakov Depot,Barratt Terminal"
+ },
+ {
+ "name": "HIP 74930",
+ "pos_x": -24.59375,
+ "pos_y": 120.21875,
+ "pos_z": 94.34375,
+ "stations": "Northrop City,Evans Hangar,Musabayev Keep"
+ },
+ {
+ "name": "HIP 74931",
+ "pos_x": -22.53125,
+ "pos_y": 110.125,
+ "pos_z": 86.4375,
+ "stations": "Morgan Dock,Abnett Arsenal"
+ },
+ {
+ "name": "HIP 74948",
+ "pos_x": -0.6875,
+ "pos_y": 105.03125,
+ "pos_z": 109.71875,
+ "stations": "Roberts Arena,Kornbluth Enterprise"
+ },
+ {
+ "name": "HIP 75011",
+ "pos_x": -57.28125,
+ "pos_y": 115.59375,
+ "pos_z": 48,
+ "stations": "Lloyd Dock,Aldiss Platform,Stevin Depot"
+ },
+ {
+ "name": "HIP 75021",
+ "pos_x": -38.875,
+ "pos_y": 132.0625,
+ "pos_z": 89.6875,
+ "stations": "Shawl Installation"
+ },
+ {
+ "name": "HIP 75024",
+ "pos_x": -109.875,
+ "pos_y": 143.875,
+ "pos_z": 9.5,
+ "stations": "Hilbert Landing"
+ },
+ {
+ "name": "HIP 75038",
+ "pos_x": 12.90625,
+ "pos_y": 95.90625,
+ "pos_z": 119.1875,
+ "stations": "Yurchikhin Terminal,Feynman Platform"
+ },
+ {
+ "name": "HIP 75039",
+ "pos_x": -68.1875,
+ "pos_y": 111.21875,
+ "pos_z": 29.4375,
+ "stations": "Young Terminal,Shelley Terminal"
+ },
+ {
+ "name": "HIP 75122",
+ "pos_x": 82.96875,
+ "pos_y": 20.25,
+ "pos_z": 131.125,
+ "stations": "Guidoni Colony"
+ },
+ {
+ "name": "HIP 75124",
+ "pos_x": -52.34375,
+ "pos_y": 124.15625,
+ "pos_z": 65.1875,
+ "stations": "Quinn Station,Bailey Point,Svavarsson Gateway,Henslow Platform,Monge Camp"
+ },
+ {
+ "name": "HIP 75132",
+ "pos_x": -47.09375,
+ "pos_y": 111.59375,
+ "pos_z": 58.59375,
+ "stations": "Tall Stop"
+ },
+ {
+ "name": "HIP 75218",
+ "pos_x": -134.875,
+ "pos_y": 130.03125,
+ "pos_z": -36.46875,
+ "stations": "Gentle Relay"
+ },
+ {
+ "name": "HIP 7526",
+ "pos_x": 52.625,
+ "pos_y": -211.5625,
+ "pos_z": -8.71875,
+ "stations": "Kulin Station,Lassell Relay"
+ },
+ {
+ "name": "HIP 75262",
+ "pos_x": -2.21875,
+ "pos_y": 94.46875,
+ "pos_z": 101,
+ "stations": "Ochoa Terminal,Carr Enterprise,Szebehely City"
+ },
+ {
+ "name": "HIP 75281",
+ "pos_x": -30.5625,
+ "pos_y": 115.09375,
+ "pos_z": 86.25,
+ "stations": "Stokes Settlement,Cramer Horizons"
+ },
+ {
+ "name": "HIP 75389",
+ "pos_x": -117.8125,
+ "pos_y": 130.53125,
+ "pos_z": -11.75,
+ "stations": "Grego Settlement,Alenquer Settlement,Rothman Survey"
+ },
+ {
+ "name": "HIP 754",
+ "pos_x": -125.25,
+ "pos_y": -99.65625,
+ "pos_z": -50.15625,
+ "stations": "Freycinet Mines"
+ },
+ {
+ "name": "HIP 75426",
+ "pos_x": 7.09375,
+ "pos_y": 89.3125,
+ "pos_z": 110.125,
+ "stations": "Born Mine"
+ },
+ {
+ "name": "HIP 75446",
+ "pos_x": -45.375,
+ "pos_y": 118.25,
+ "pos_z": 72.34375,
+ "stations": "Crowley Enterprise,Weiss Hub,Shaara Beacon"
+ },
+ {
+ "name": "HIP 7564",
+ "pos_x": -80.28125,
+ "pos_y": -85.34375,
+ "pos_z": -84.875,
+ "stations": "Stewart Orbital,Thoreau Landing,Gantt Mines,Scheerbart's Inheritance,Bayley's Folly,Reilly Point"
+ },
+ {
+ "name": "HIP 75762",
+ "pos_x": 19.21875,
+ "pos_y": 68,
+ "pos_z": 106.78125,
+ "stations": "Shapiro Gateway,Garrett Ring,Harding Depot,Birkhoff's Claim,Stabenow Terminal,Gloss Survey,Slonczewski Base"
+ },
+ {
+ "name": "HIP 75862",
+ "pos_x": -94.6875,
+ "pos_y": 163.28125,
+ "pos_z": 63.8125,
+ "stations": "Rond d'Alembert Mines,Panshin's Inheritance"
+ },
+ {
+ "name": "HIP 75964",
+ "pos_x": -25.21875,
+ "pos_y": 119.21875,
+ "pos_z": 109.21875,
+ "stations": "Sternberg Dock,Brackett Depot,Fourier Arena"
+ },
+ {
+ "name": "HIP 75978",
+ "pos_x": -41.1875,
+ "pos_y": 114.875,
+ "pos_z": 81.78125,
+ "stations": "Bondar Orbital,Ham Colony,Pausch Horizons"
+ },
+ {
+ "name": "HIP 76045",
+ "pos_x": -95.53125,
+ "pos_y": 130.15625,
+ "pos_z": 25.15625,
+ "stations": "Kennan Installation,McMullen Base"
+ },
+ {
+ "name": "HIP 76058",
+ "pos_x": -19.3125,
+ "pos_y": 117.25,
+ "pos_z": 116.84375,
+ "stations": "Hudson Ring,Lerner Station,Lichtenberg Depot"
+ },
+ {
+ "name": "HIP 76156",
+ "pos_x": -89.9375,
+ "pos_y": 132.65625,
+ "pos_z": 37.71875,
+ "stations": "Atiyah Terminal,Steakley Port,Marley Exchange"
+ },
+ {
+ "name": "HIP 76189",
+ "pos_x": -64.75,
+ "pos_y": 143.6875,
+ "pos_z": 87.9375,
+ "stations": "Bergerac Point,Herreshoff Reach,Rocklynne Enterprise"
+ },
+ {
+ "name": "HIP 76203",
+ "pos_x": 19.15625,
+ "pos_y": 63.5625,
+ "pos_z": 107.28125,
+ "stations": "Pettit Terminal,Lobachevsky Beacon,Potrykus Hub,Bagian Port,Perry Plant"
+ },
+ {
+ "name": "HIP 76256",
+ "pos_x": -113.34375,
+ "pos_y": 107.21875,
+ "pos_z": -26.46875,
+ "stations": "Amundsen Platform,Serling Orbital,Schiltberger Laboratory"
+ },
+ {
+ "name": "HIP 76280",
+ "pos_x": -22.125,
+ "pos_y": 99.40625,
+ "pos_z": 94.59375,
+ "stations": "Lind Terminal,Lonchakov Dock,Cseszneky Gateway,Tevis Base,Siodmak Silo"
+ },
+ {
+ "name": "HIP 76282",
+ "pos_x": -34.125,
+ "pos_y": 104,
+ "pos_z": 83.28125,
+ "stations": "Porsche Relay"
+ },
+ {
+ "name": "HIP 76292",
+ "pos_x": -3.90625,
+ "pos_y": 86,
+ "pos_z": 104.03125,
+ "stations": "Melvin Terminal,Coles Depot,Griffith Legacy"
+ },
+ {
+ "name": "HIP 76398",
+ "pos_x": -83.15625,
+ "pos_y": 135.15625,
+ "pos_z": 54.21875,
+ "stations": "Sverdrup Dock,Bohnhoff Platform,Palmer Hub"
+ },
+ {
+ "name": "HIP 76435",
+ "pos_x": 70.09375,
+ "pos_y": 28.6875,
+ "pos_z": 139.09375,
+ "stations": "Jordan Terminal,Grant Installation"
+ },
+ {
+ "name": "HIP 76466",
+ "pos_x": -128.15625,
+ "pos_y": 127.15625,
+ "pos_z": -20.6875,
+ "stations": "Budrys Station,George Platform,Broderick Arsenal,Quaglia City"
+ },
+ {
+ "name": "HIP 76543",
+ "pos_x": -31,
+ "pos_y": 106.75,
+ "pos_z": 95.4375,
+ "stations": "Smith Hub,Mallett Terminal,Perga Terminal,Diophantus Terminal,Balmer Vision,Godel Port"
+ },
+ {
+ "name": "HIP 76629",
+ "pos_x": 73.5,
+ "pos_y": -4.9375,
+ "pos_z": 101.8125,
+ "stations": "Holland Station,Maury Hub,Wetherill Enterprise,Taylor Holdings"
+ },
+ {
+ "name": "HIP 76635",
+ "pos_x": -14.46875,
+ "pos_y": 118.59375,
+ "pos_z": 137.15625,
+ "stations": "Narita Enterprise,Spassky Terminal"
+ },
+ {
+ "name": "HIP 76650",
+ "pos_x": 65.375,
+ "pos_y": 25.03125,
+ "pos_z": 130.0625,
+ "stations": "Potter Mine,Glidden Holdings"
+ },
+ {
+ "name": "HIP 7670",
+ "pos_x": -124.6875,
+ "pos_y": 4.5625,
+ "pos_z": -99.125,
+ "stations": "McMahon Colony,Moore Hub"
+ },
+ {
+ "name": "HIP 76701",
+ "pos_x": 46.78125,
+ "pos_y": 41.65625,
+ "pos_z": 125.4375,
+ "stations": "Rubruck Dock"
+ },
+ {
+ "name": "HIP 76713",
+ "pos_x": 99.625,
+ "pos_y": -14.5,
+ "pos_z": 128.28125,
+ "stations": "Erdos Base"
+ },
+ {
+ "name": "HIP 7674",
+ "pos_x": 106.84375,
+ "pos_y": -213.46875,
+ "pos_z": 32.46875,
+ "stations": "Goodman Enterprise,Parsons Orbital,Bothezat Survey"
+ },
+ {
+ "name": "HIP 76747",
+ "pos_x": 104.0625,
+ "pos_y": -35.40625,
+ "pos_z": 106.875,
+ "stations": "Denning Vision,Frobisher Lab,Robert Port"
+ },
+ {
+ "name": "HIP 76768",
+ "pos_x": 20.375,
+ "pos_y": 61.4375,
+ "pos_z": 113.96875,
+ "stations": "Bethe Orbital,Jemison City,Vasyutin Hub"
+ },
+ {
+ "name": "HIP 76837",
+ "pos_x": -125.21875,
+ "pos_y": 131.1875,
+ "pos_z": -7.40625,
+ "stations": "Zettel Station,Menzies Port,Turzillo Orbital,MacLean Horizons"
+ },
+ {
+ "name": "HIP 7687",
+ "pos_x": 13.96875,
+ "pos_y": -124.125,
+ "pos_z": -19.90625,
+ "stations": "Oikawa Point"
+ },
+ {
+ "name": "HIP 76911",
+ "pos_x": -76.6875,
+ "pos_y": 134.78125,
+ "pos_z": 71.6875,
+ "stations": "Bamford Horizons"
+ },
+ {
+ "name": "HIP 76954",
+ "pos_x": 24,
+ "pos_y": 103.0625,
+ "pos_z": 180.46875,
+ "stations": "Resnick Mine,Gresh Dock,Sutcliffe Refinery"
+ },
+ {
+ "name": "HIP 76982",
+ "pos_x": 12.5625,
+ "pos_y": 87.28125,
+ "pos_z": 141.6875,
+ "stations": "Hugh Horizons,Quaglia Terminal"
+ },
+ {
+ "name": "HIP 7705",
+ "pos_x": -123.03125,
+ "pos_y": -53.03125,
+ "pos_z": -112.46875,
+ "stations": "Alexandria Colony,Salak Relay"
+ },
+ {
+ "name": "HIP 77083",
+ "pos_x": -44.0625,
+ "pos_y": 111.53125,
+ "pos_z": 91.625,
+ "stations": "Dzhanibekov Station,Dunn Station,Hui Barracks"
+ },
+ {
+ "name": "HIP 77129",
+ "pos_x": -69.0625,
+ "pos_y": 143.96875,
+ "pos_z": 100.625,
+ "stations": "Starzl Terminal,Compton Depot,Cauchy Prospect,Ehrenfried Kegel Platform"
+ },
+ {
+ "name": "HIP 77199",
+ "pos_x": 37.8125,
+ "pos_y": 41.6875,
+ "pos_z": 118.96875,
+ "stations": "Belyanin Terminal,King Hub,Snodgrass Dock,Coulomb Enterprise,Mitchell Hub"
+ },
+ {
+ "name": "HIP 772",
+ "pos_x": 4.875,
+ "pos_y": -189.375,
+ "pos_z": 35.78125,
+ "stations": "Bowen Orbital,Tombaugh Station,Cowell Station,Wallis Keep,Anderson Installation,Minkowski Hub,Hague Camp"
+ },
+ {
+ "name": "HIP 77282",
+ "pos_x": 66.15625,
+ "pos_y": 22.375,
+ "pos_z": 135.84375,
+ "stations": "Evason Landing"
+ },
+ {
+ "name": "HIP 77310",
+ "pos_x": 80.46875,
+ "pos_y": -15.75,
+ "pos_z": 102.375,
+ "stations": "Grabe Hangar"
+ },
+ {
+ "name": "HIP 77451",
+ "pos_x": -77.15625,
+ "pos_y": 101.375,
+ "pos_z": 30.71875,
+ "stations": "Low's Claim,Faraday Penal colony"
+ },
+ {
+ "name": "HIP 77470",
+ "pos_x": 140.5625,
+ "pos_y": -81.84375,
+ "pos_z": 98.9375,
+ "stations": "Chelomey Orbital,Herschel Station,Wachmann Station,Shiner Settlement,Hiraga Relay"
+ },
+ {
+ "name": "HIP 77534",
+ "pos_x": 17.1875,
+ "pos_y": 65.125,
+ "pos_z": 126.8125,
+ "stations": "Edison Relay,Cameron Refinery,Stabenow Keep"
+ },
+ {
+ "name": "HIP 7759",
+ "pos_x": -19.84375,
+ "pos_y": -149.5,
+ "pos_z": -54.34375,
+ "stations": "Hyecho Vision,Mayer City,Gehry Dock,Struzan Colony"
+ },
+ {
+ "name": "HIP 77610",
+ "pos_x": 33.1875,
+ "pos_y": 69.53125,
+ "pos_z": 160.375,
+ "stations": "Lem Terminal,Saavedra Landing,Ferguson Colony,Pashin Arsenal"
+ },
+ {
+ "name": "HIP 7766",
+ "pos_x": 97,
+ "pos_y": -205.5625,
+ "pos_z": 25.65625,
+ "stations": "Herschel Terminal,Fiorilla Colony,Robert's Folly"
+ },
+ {
+ "name": "HIP 77749",
+ "pos_x": -12.40625,
+ "pos_y": 76.40625,
+ "pos_z": 100.5,
+ "stations": "Linnaeus City,Boswell Ring"
+ },
+ {
+ "name": "HIP 77807",
+ "pos_x": -128,
+ "pos_y": 131.5625,
+ "pos_z": 0.46875,
+ "stations": "Roberts Dock,Wallin Horizons,Sekowski Horizons,Maler Beacon,Lloyd Terminal"
+ },
+ {
+ "name": "HIP 77818",
+ "pos_x": 96.03125,
+ "pos_y": -17.625,
+ "pos_z": 128.96875,
+ "stations": "Walker Orbital,Francisco de Eliza Keep,Bosch Orbital"
+ },
+ {
+ "name": "HIP 77838",
+ "pos_x": -117.375,
+ "pos_y": 103.46875,
+ "pos_z": -26.6875,
+ "stations": "Byrd Terminal"
+ },
+ {
+ "name": "HIP 7799",
+ "pos_x": -21.3125,
+ "pos_y": -171.65625,
+ "pos_z": -61.71875,
+ "stations": "Lessing Port,Platt Terminal,Cobb Keep"
+ },
+ {
+ "name": "HIP 78074",
+ "pos_x": -81,
+ "pos_y": 110.25,
+ "pos_z": 47.3125,
+ "stations": "Sargent City,Hendel Ring,Bachman Relay,Brooks Landing"
+ },
+ {
+ "name": "HIP 78136",
+ "pos_x": 53.8125,
+ "pos_y": 62.09375,
+ "pos_z": 194.875,
+ "stations": "Garden Enterprise,Shiras Enterprise,Dietz Vision,Strzelecki Enterprise"
+ },
+ {
+ "name": "HIP 78139",
+ "pos_x": -84.4375,
+ "pos_y": 119.90625,
+ "pos_z": 58.84375,
+ "stations": "Britnev Hub"
+ },
+ {
+ "name": "HIP 78158",
+ "pos_x": -71.28125,
+ "pos_y": 101.03125,
+ "pos_z": 49.65625,
+ "stations": "Hartlib Depot,Lander Oasis"
+ },
+ {
+ "name": "HIP 78165",
+ "pos_x": -116.25,
+ "pos_y": 106.03125,
+ "pos_z": -17.84375,
+ "stations": "Hand Dock,Lobachevsky Landing,Bramah Survey"
+ },
+ {
+ "name": "HIP 7817",
+ "pos_x": 23.46875,
+ "pos_y": -142.375,
+ "pos_z": -18.03125,
+ "stations": "Suydam Dock,Cerulli Market,van de Sande Bakhuyzen Station,Naylor Dock,Fallows Station,Boyer Station,Gaensler Reach,Deslandres Vision,Tank Enterprise,Merrill Terminal,Veron Arsenal"
+ },
+ {
+ "name": "HIP 7819",
+ "pos_x": -65.15625,
+ "pos_y": -140.125,
+ "pos_z": -89.25,
+ "stations": "Atiyah Lab"
+ },
+ {
+ "name": "HIP 7823",
+ "pos_x": 39.65625,
+ "pos_y": -222.03125,
+ "pos_z": -25.65625,
+ "stations": "Henderson Dock,Albategnius Dock,Escobar Horizons"
+ },
+ {
+ "name": "HIP 78237",
+ "pos_x": -115.75,
+ "pos_y": 117.28125,
+ "pos_z": 2.78125,
+ "stations": "Qian Vision"
+ },
+ {
+ "name": "HIP 78251",
+ "pos_x": 27.5,
+ "pos_y": 57.34375,
+ "pos_z": 144.71875,
+ "stations": "Normand Station,Offutt Station,Arnason Depot"
+ },
+ {
+ "name": "HIP 78267",
+ "pos_x": 43.28125,
+ "pos_y": 21.28125,
+ "pos_z": 110.34375,
+ "stations": "al-Khowarizmi's Progress,Porges Platform"
+ },
+ {
+ "name": "HIP 7830",
+ "pos_x": -60.28125,
+ "pos_y": -96.03125,
+ "pos_z": -73.9375,
+ "stations": "MacCurdy Platform,Simak Dock,North Freeport,Elwood Depot,Vasilyev Settlement"
+ },
+ {
+ "name": "HIP 78313",
+ "pos_x": 43.96875,
+ "pos_y": 49.3125,
+ "pos_z": 160.5,
+ "stations": "Vasilyev Retreat"
+ },
+ {
+ "name": "HIP 78399",
+ "pos_x": -19.375,
+ "pos_y": 78.9375,
+ "pos_z": 104.25,
+ "stations": "Cook Ring,Volterra Enterprise,Abasheli Horizons,Avogadro Horizons,Frobisher Penal colony"
+ },
+ {
+ "name": "HIP 78466",
+ "pos_x": 42.375,
+ "pos_y": 38.1875,
+ "pos_z": 141,
+ "stations": "Barnes' Claim,Pausch Vision"
+ },
+ {
+ "name": "HIP 78505",
+ "pos_x": 133.5,
+ "pos_y": -63.875,
+ "pos_z": 119.78125,
+ "stations": "Abernathy's Claim,Tsiolkovsky Mines"
+ },
+ {
+ "name": "HIP 78513",
+ "pos_x": -18,
+ "pos_y": 101.625,
+ "pos_z": 149.03125,
+ "stations": "Ziemianski Relay"
+ },
+ {
+ "name": "HIP 7852",
+ "pos_x": -30.9375,
+ "pos_y": -153.375,
+ "pos_z": -65.34375,
+ "stations": "Weaver Ring"
+ },
+ {
+ "name": "HIP 78551",
+ "pos_x": 13.96875,
+ "pos_y": 52.96875,
+ "pos_z": 119.3125,
+ "stations": "Bergerac Terminal,Herreshoff Keep,Kapp Observatory"
+ },
+ {
+ "name": "HIP 78647",
+ "pos_x": 31.1875,
+ "pos_y": 50.03125,
+ "pos_z": 145.9375,
+ "stations": "Norman Arsenal"
+ },
+ {
+ "name": "HIP 78688",
+ "pos_x": -49.78125,
+ "pos_y": 102.21875,
+ "pos_z": 98.1875,
+ "stations": "Beadle Dock,Jones Arsenal"
+ },
+ {
+ "name": "HIP 7869",
+ "pos_x": 127.6875,
+ "pos_y": -162.5,
+ "pos_z": 60.84375,
+ "stations": "Malvern Dock,Seares Orbital"
+ },
+ {
+ "name": "HIP 787",
+ "pos_x": -1.25,
+ "pos_y": -130.4375,
+ "pos_z": 22.03125,
+ "stations": "Neujmin Settlement,Takahashi Vision,Regiomontanus Station,Coande Port,Searle Dock,Nijland Installation,Proctor Terminal,Kotelnikov's Pride,Chernykh Station,Ziegler Orbital,Friedrich Peters Orbital,Regiomontanus Hub,Barton Depot,McKee Depot"
+ },
+ {
+ "name": "HIP 78704",
+ "pos_x": 17.78125,
+ "pos_y": 73.6875,
+ "pos_z": 166.6875,
+ "stations": "Beebe Prospect"
+ },
+ {
+ "name": "HIP 78716",
+ "pos_x": 42.5,
+ "pos_y": 24.15625,
+ "pos_z": 120.21875,
+ "stations": "Prunariu Port"
+ },
+ {
+ "name": "HIP 78864",
+ "pos_x": -40.375,
+ "pos_y": 89.375,
+ "pos_z": 94.875,
+ "stations": "Makarov Settlement"
+ },
+ {
+ "name": "HIP 78866",
+ "pos_x": 194.84375,
+ "pos_y": -118.9375,
+ "pos_z": 129.375,
+ "stations": "Laming Depot"
+ },
+ {
+ "name": "HIP 78883",
+ "pos_x": -2,
+ "pos_y": 58.65625,
+ "pos_z": 107,
+ "stations": "Bixby Depot,Bentham Plant,Maire Holdings"
+ },
+ {
+ "name": "HIP 78923",
+ "pos_x": -83.125,
+ "pos_y": 110.8125,
+ "pos_z": 58.875,
+ "stations": "Antonio de Andrade Terminal,Taylor Estate"
+ },
+ {
+ "name": "HIP 78947",
+ "pos_x": 17.375,
+ "pos_y": 43.5625,
+ "pos_z": 114.84375,
+ "stations": "Pausch Port"
+ },
+ {
+ "name": "HIP 78983",
+ "pos_x": -6.875,
+ "pos_y": 60.75,
+ "pos_z": 103.8125,
+ "stations": "Coulter Vista,Marques Dock,Pavlou Terminal,Rothman Orbital,Shumil Terminal,Lowry Point,Koontz Port,Farouk Hub,Carlisle Orbital,Disch Terminal,Crown Platform,Sauma's Folly,Freycinet Mines,Napier Lab,Nakaya Installation"
+ },
+ {
+ "name": "HIP 79008",
+ "pos_x": -25.59375,
+ "pos_y": 88.6875,
+ "pos_z": 123.59375,
+ "stations": "Borisenko Silo,von Helmont City,Meyrink Works"
+ },
+ {
+ "name": "HIP 79067",
+ "pos_x": 88.78125,
+ "pos_y": -15.03125,
+ "pos_z": 135.15625,
+ "stations": "Banks Refinery"
+ },
+ {
+ "name": "HIP 791",
+ "pos_x": 32.5625,
+ "pos_y": -241.53125,
+ "pos_z": 59.625,
+ "stations": "Johnson Works"
+ },
+ {
+ "name": "HIP 79117",
+ "pos_x": -31.96875,
+ "pos_y": 96.90625,
+ "pos_z": 130.34375,
+ "stations": "Kooi Arsenal,Savery Station,Qureshi Platform,Bohr Orbital"
+ },
+ {
+ "name": "HIP 79152",
+ "pos_x": -75.3125,
+ "pos_y": 89.21875,
+ "pos_z": 35.125,
+ "stations": "Makarov City,Mallett Installation,Elder City"
+ },
+ {
+ "name": "HIP 7916",
+ "pos_x": -12.9375,
+ "pos_y": -120.6875,
+ "pos_z": -42.71875,
+ "stations": "Froud Vision,Giunta Gateway,Miller Ring,Janjetov Gateway,Wolszczan Orbital,Gill Port,Dahm Ring,Fraknoi Hub,Axon Gateway"
+ },
+ {
+ "name": "HIP 79234",
+ "pos_x": -109.6875,
+ "pos_y": 130.09375,
+ "pos_z": 53.03125,
+ "stations": "Stevens Horizons,Doyle Enterprise"
+ },
+ {
+ "name": "HIP 7924",
+ "pos_x": -124.75,
+ "pos_y": -49.90625,
+ "pos_z": -114.9375,
+ "stations": "Bunch Hub,Kingsbury Settlement,Murphy Silo"
+ },
+ {
+ "name": "HIP 79276",
+ "pos_x": -99.46875,
+ "pos_y": 92.90625,
+ "pos_z": -1.40625,
+ "stations": "Hobaugh Enterprise,Northrop Station,Herreshoff Keep,Guest Hub,Heaviside Installation,Beatty Installation"
+ },
+ {
+ "name": "HIP 7930",
+ "pos_x": -64.9375,
+ "pos_y": -97.90625,
+ "pos_z": -79.15625,
+ "stations": "Rashid Settlement"
+ },
+ {
+ "name": "HIP 79308",
+ "pos_x": -85.0625,
+ "pos_y": 91.78125,
+ "pos_z": 23.90625,
+ "stations": "Boas Hub,Gohar Platform,Sakers Survey"
+ },
+ {
+ "name": "HIP 79315",
+ "pos_x": 90.53125,
+ "pos_y": -19.75,
+ "pos_z": 132,
+ "stations": "Zholobov Port,Wolf Dock,Zindell Depot,Gulyaev Installation,Zhen Park"
+ },
+ {
+ "name": "HIP 79322",
+ "pos_x": 49.9375,
+ "pos_y": 8.46875,
+ "pos_z": 112.09375,
+ "stations": "Torricelli Ring,Shepard Terminal,Aleksandrov Station,Kent Keep,Bunnell Horizons"
+ },
+ {
+ "name": "HIP 79327",
+ "pos_x": -71.53125,
+ "pos_y": 100.375,
+ "pos_z": 67.34375,
+ "stations": "Robinson Orbital"
+ },
+ {
+ "name": "HIP 794",
+ "pos_x": 3.78125,
+ "pos_y": -177.34375,
+ "pos_z": 32.84375,
+ "stations": "Hume Prospect,von Zach Station,Iben Vision,Mori Hub,Loncke Relay"
+ },
+ {
+ "name": "HIP 79419",
+ "pos_x": 44.5625,
+ "pos_y": 21.9375,
+ "pos_z": 130.78125,
+ "stations": "Herschel Platform,Zamka Dock,Birmingham Vision"
+ },
+ {
+ "name": "HIP 7949",
+ "pos_x": -117.5625,
+ "pos_y": 18.15625,
+ "pos_z": -91,
+ "stations": "Onnes Mines,Walker Camp"
+ },
+ {
+ "name": "HIP 79576",
+ "pos_x": 62.125,
+ "pos_y": -0.75,
+ "pos_z": 119.65625,
+ "stations": "Snyder Hub,Gillekens Hub,Baudry Terminal,Nicollet Terminal"
+ },
+ {
+ "name": "HIP 79594",
+ "pos_x": -60.59375,
+ "pos_y": 94.8125,
+ "pos_z": 81.84375,
+ "stations": "Binet City,Bolotov Horizons,Marshall Terminal"
+ },
+ {
+ "name": "HIP 7961",
+ "pos_x": -1.46875,
+ "pos_y": -149.75,
+ "pos_z": -41.5625,
+ "stations": "Banno Station,Dubyago Dock,Matthews Ring,Pollas' Claim"
+ },
+ {
+ "name": "HIP 79619",
+ "pos_x": -99.3125,
+ "pos_y": 79.96875,
+ "pos_z": -24.90625,
+ "stations": "Barnes Depot,Kooi City,Coats Dock,Carson Settlement"
+ },
+ {
+ "name": "HIP 79636",
+ "pos_x": 69.46875,
+ "pos_y": -10.40625,
+ "pos_z": 114.59375,
+ "stations": "Nakamura City,Haro Orbital,Zubrin Vision,Kovalevskaya Point"
+ },
+ {
+ "name": "HIP 79682",
+ "pos_x": 117.9375,
+ "pos_y": -65.65625,
+ "pos_z": 92.25,
+ "stations": "Riazuddin Ring,Fowler Vision,Siddha Dock"
+ },
+ {
+ "name": "HIP 79730",
+ "pos_x": -26.03125,
+ "pos_y": 82.78125,
+ "pos_z": 128.0625,
+ "stations": "Ziemkiewicz Colony,Riemann Prospect,Klein Holdings"
+ },
+ {
+ "name": "HIP 79735",
+ "pos_x": 93.875,
+ "pos_y": -29.40625,
+ "pos_z": 123.25,
+ "stations": "Gresley Observatory,Fearn Port,Aldiss Dock"
+ },
+ {
+ "name": "HIP 79884",
+ "pos_x": 60.15625,
+ "pos_y": 33.875,
+ "pos_z": 198.1875,
+ "stations": "Hartog Prospect,Cartan Base"
+ },
+ {
+ "name": "HIP 79888",
+ "pos_x": 58.5,
+ "pos_y": 13.875,
+ "pos_z": 150.09375,
+ "stations": "Maclaurin Enterprise,Perry Camp"
+ },
+ {
+ "name": "HIP 79969",
+ "pos_x": 18.21875,
+ "pos_y": 35.90625,
+ "pos_z": 119.09375,
+ "stations": "Ahmed Terminal,Gelfand Gateway,Anderson Dock"
+ },
+ {
+ "name": "HIP 79981",
+ "pos_x": -105,
+ "pos_y": 83.96875,
+ "pos_z": -24.6875,
+ "stations": "Sverdrup Colony,Grant Point"
+ },
+ {
+ "name": "HIP 80065",
+ "pos_x": 57.84375,
+ "pos_y": 6.375,
+ "pos_z": 135.03125,
+ "stations": "Cayley Station,Card Station"
+ },
+ {
+ "name": "HIP 80096",
+ "pos_x": -58.28125,
+ "pos_y": 87.28125,
+ "pos_z": 81.625,
+ "stations": "Sommerfeld Hangar,Franklin Terminal,Gardner Dock,Davis Terminal"
+ },
+ {
+ "name": "HIP 801",
+ "pos_x": 47.28125,
+ "pos_y": -159.8125,
+ "pos_z": 53.375,
+ "stations": "Potocnik Gateway,Kirby Ring,Theodor Winnecke Terminal,Weaver Port,Donati Station,Sikorsky Hub,Folland Orbital"
+ },
+ {
+ "name": "HIP 80122",
+ "pos_x": -123.90625,
+ "pos_y": 93.96875,
+ "pos_z": -39.75,
+ "stations": "Mitchell Dock,Citi Base,Baxter Relay"
+ },
+ {
+ "name": "HIP 80162",
+ "pos_x": -54.96875,
+ "pos_y": 108.46875,
+ "pos_z": 139.96875,
+ "stations": "Serrao Hub,McDonald Terminal,Stefansson Enterprise"
+ },
+ {
+ "name": "HIP 80182",
+ "pos_x": -60.65625,
+ "pos_y": 104.1875,
+ "pos_z": 118.53125,
+ "stations": "Eyharts Landing,Porsche Port,Chadwick Depot"
+ },
+ {
+ "name": "HIP 80221",
+ "pos_x": 60.15625,
+ "pos_y": -7.53125,
+ "pos_z": 109.8125,
+ "stations": "Somerset Port,Griggs City,Coles Barracks"
+ },
+ {
+ "name": "HIP 80232",
+ "pos_x": -121.65625,
+ "pos_y": 127.375,
+ "pos_z": 45.84375,
+ "stations": "Barnes Prospect,Janes Oasis"
+ },
+ {
+ "name": "HIP 80242",
+ "pos_x": 25.125,
+ "pos_y": 26.1875,
+ "pos_z": 116.34375,
+ "stations": "Csoma Ring,Cockrell Base,Frobisher Silo"
+ },
+ {
+ "name": "HIP 80243",
+ "pos_x": -92.78125,
+ "pos_y": 80.625,
+ "pos_z": -4.375,
+ "stations": "Detmer Ring,Alas Port,Yegorov Terminal"
+ },
+ {
+ "name": "HIP 80250",
+ "pos_x": 78,
+ "pos_y": -21.6875,
+ "pos_z": 114.34375,
+ "stations": "Cixin Mines,Moore Enterprise,Galindo Vision"
+ },
+ {
+ "name": "HIP 80266",
+ "pos_x": -34.28125,
+ "pos_y": 87.8125,
+ "pos_z": 137.9375,
+ "stations": "Cavalieri Dock,Reamy Installation,Janes Barracks"
+ },
+ {
+ "name": "HIP 80273",
+ "pos_x": -34.5,
+ "pos_y": 90.25,
+ "pos_z": 143.40625,
+ "stations": "Liouville Barracks,Cixin Beacon"
+ },
+ {
+ "name": "HIP 80364",
+ "pos_x": -101.59375,
+ "pos_y": 93.78125,
+ "pos_z": 9.90625,
+ "stations": "Stasheff Colony,Moorcock Arsenal,Birmingham Orbital"
+ },
+ {
+ "name": "HIP 80608",
+ "pos_x": -16,
+ "pos_y": 78.8125,
+ "pos_z": 167.1875,
+ "stations": "Tombaugh Base"
+ },
+ {
+ "name": "HIP 80616",
+ "pos_x": -25.53125,
+ "pos_y": 70.875,
+ "pos_z": 125.65625,
+ "stations": "Szameit Plant,Cortes Refinery"
+ },
+ {
+ "name": "HIP 80680",
+ "pos_x": 64.71875,
+ "pos_y": 3.4375,
+ "pos_z": 155.3125,
+ "stations": "Caryanda Mines,Arnason Enterprise,Alvares Colony"
+ },
+ {
+ "name": "HIP 80700",
+ "pos_x": -40.96875,
+ "pos_y": 82.625,
+ "pos_z": 123.59375,
+ "stations": "Vries Orbital"
+ },
+ {
+ "name": "HIP 80706",
+ "pos_x": 71.40625,
+ "pos_y": -16.375,
+ "pos_z": 119.25,
+ "stations": "Carey Landing"
+ },
+ {
+ "name": "HIP 80742",
+ "pos_x": -136.3125,
+ "pos_y": 116.78125,
+ "pos_z": -2.53125,
+ "stations": "Lanier Dock"
+ },
+ {
+ "name": "HIP 80818",
+ "pos_x": -121.4375,
+ "pos_y": 117,
+ "pos_z": 34.09375,
+ "stations": "Robson's Progress"
+ },
+ {
+ "name": "HIP 80868",
+ "pos_x": 18.375,
+ "pos_y": 27.3125,
+ "pos_z": 117,
+ "stations": "Wul Survey"
+ },
+ {
+ "name": "HIP 80870",
+ "pos_x": 25.78125,
+ "pos_y": 38.3125,
+ "pos_z": 164.09375,
+ "stations": "Ron Hubbard's Claim"
+ },
+ {
+ "name": "HIP 80936",
+ "pos_x": -61.1875,
+ "pos_y": 93.03125,
+ "pos_z": 112.34375,
+ "stations": "Caillie Retreat"
+ },
+ {
+ "name": "HIP 81010",
+ "pos_x": -3,
+ "pos_y": 50.8125,
+ "pos_z": 135.25,
+ "stations": "Polansky Hub,Stafford Hub,Mendeleev Terminal,Evans Hub"
+ },
+ {
+ "name": "HIP 81046",
+ "pos_x": -26.0625,
+ "pos_y": 77.65625,
+ "pos_z": 157.1875,
+ "stations": "Readdy Orbital,Salam Horizons"
+ },
+ {
+ "name": "HIP 81061",
+ "pos_x": -91.65625,
+ "pos_y": 110.40625,
+ "pos_z": 92.90625,
+ "stations": "Conway Installation,Gloss Depot"
+ },
+ {
+ "name": "HIP 81071",
+ "pos_x": 57.75,
+ "pos_y": 2.375,
+ "pos_z": 145.75,
+ "stations": "Bester Base,Lazutkin Point,Foale Landing"
+ },
+ {
+ "name": "HIP 81085",
+ "pos_x": -117.78125,
+ "pos_y": 122.59375,
+ "pos_z": 65.5625,
+ "stations": "Illy Laboratory,Weston Dock,Nakaya Mines"
+ },
+ {
+ "name": "HIP 8109",
+ "pos_x": -108.0625,
+ "pos_y": -17.21875,
+ "pos_z": -93.75,
+ "stations": "Beebe Dock,Nicollet Gateway,Hahn Platform,Budarin Silo"
+ },
+ {
+ "name": "HIP 81177",
+ "pos_x": -81.03125,
+ "pos_y": 101.53125,
+ "pos_z": 97.09375,
+ "stations": "Ingstad's Pride"
+ },
+ {
+ "name": "HIP 81186",
+ "pos_x": -93.25,
+ "pos_y": 82.375,
+ "pos_z": 11.5625,
+ "stations": "Eckford Orbital,Balmer Station,Copernicus Prospect,Alten Prospect,Rayhan al-Biruni City,Steinmuller City"
+ },
+ {
+ "name": "HIP 8119",
+ "pos_x": 162.125,
+ "pos_y": -138.96875,
+ "pos_z": 94.375,
+ "stations": "Seitter City,Suntzeff Station,Zel'dovich Ring,Matthews Relay,Reynolds Lab"
+ },
+ {
+ "name": "HIP 81223",
+ "pos_x": -74.4375,
+ "pos_y": 116.53125,
+ "pos_z": 158.78125,
+ "stations": "Meaney Terminal"
+ },
+ {
+ "name": "HIP 81237",
+ "pos_x": 1.125,
+ "pos_y": 40.96875,
+ "pos_z": 123.34375,
+ "stations": "Song Terminal,Chang-Diaz Dock,Banks Hub,Aleksandrov Terminal,Vesalius Station,Crick Station,Land Gateway,Forrester Port,Khan Port,Haber Port,Ingstad Exchange,Kimberlin Depot"
+ },
+ {
+ "name": "HIP 81269",
+ "pos_x": 48.3125,
+ "pos_y": 20.15625,
+ "pos_z": 179.625,
+ "stations": "Gardner Platform,Acharya's Claim"
+ },
+ {
+ "name": "HIP 81294",
+ "pos_x": -5.5,
+ "pos_y": 46.5,
+ "pos_z": 124.9375,
+ "stations": "Shelley Sanctuary"
+ },
+ {
+ "name": "HIP 81335",
+ "pos_x": 45.9375,
+ "pos_y": 23.65625,
+ "pos_z": 186.15625,
+ "stations": "Crook Enterprise"
+ },
+ {
+ "name": "HIP 81353",
+ "pos_x": 95.28125,
+ "pos_y": -36.25,
+ "pos_z": 130.21875,
+ "stations": "Andreas Vision"
+ },
+ {
+ "name": "HIP 81371",
+ "pos_x": 77.09375,
+ "pos_y": -18.71875,
+ "pos_z": 137.625,
+ "stations": "Sakers Landing,Sarmiento de Gamboa Beacon,King Horizons"
+ },
+ {
+ "name": "HIP 81378",
+ "pos_x": -78.25,
+ "pos_y": 85.34375,
+ "pos_z": 61.25,
+ "stations": "Morris Ring"
+ },
+ {
+ "name": "HIP 81390",
+ "pos_x": 104.28125,
+ "pos_y": -59.03125,
+ "pos_z": 84.1875,
+ "stations": "Lalande Depot,Argelander Camp"
+ },
+ {
+ "name": "HIP 81469",
+ "pos_x": 68.71875,
+ "pos_y": -16.71875,
+ "pos_z": 124.46875,
+ "stations": "Corbusier City,Haack Port"
+ },
+ {
+ "name": "HIP 81498",
+ "pos_x": 23.71875,
+ "pos_y": 28.625,
+ "pos_z": 150.46875,
+ "stations": "Xuanzang Hub"
+ },
+ {
+ "name": "HIP 81650",
+ "pos_x": 68.09375,
+ "pos_y": -10.71875,
+ "pos_z": 145.625,
+ "stations": "Offutt Station,Popov Terminal"
+ },
+ {
+ "name": "HIP 81662",
+ "pos_x": -76.96875,
+ "pos_y": 90.5625,
+ "pos_z": 89.625,
+ "stations": "McCoy Enterprise"
+ },
+ {
+ "name": "HIP 81755",
+ "pos_x": -132.03125,
+ "pos_y": 104.4375,
+ "pos_z": -10.3125,
+ "stations": "Noon Landing"
+ },
+ {
+ "name": "HIP 81767",
+ "pos_x": 22.875,
+ "pos_y": 31.15625,
+ "pos_z": 165.21875,
+ "stations": "Belyayev Dock,Hornby Relay"
+ },
+ {
+ "name": "HIP 81831",
+ "pos_x": -68,
+ "pos_y": 79.9375,
+ "pos_z": 83.5,
+ "stations": "Hilbert Port,Vlamingh Station"
+ },
+ {
+ "name": "HIP 81862",
+ "pos_x": -96.3125,
+ "pos_y": 79.03125,
+ "pos_z": 3.65625,
+ "stations": "Bachman Dock,Simmons Keep"
+ },
+ {
+ "name": "HIP 81896",
+ "pos_x": 40.59375,
+ "pos_y": 9.59375,
+ "pos_z": 144.96875,
+ "stations": "Arnason Orbital,Bulmer Port,Sawyer Enterprise"
+ },
+ {
+ "name": "HIP 81910",
+ "pos_x": 15.03125,
+ "pos_y": 30.875,
+ "pos_z": 147.53125,
+ "stations": "Wang Hangar,Brahe Station"
+ },
+ {
+ "name": "HIP 8193",
+ "pos_x": 121.03125,
+ "pos_y": -216,
+ "pos_z": 38.0625,
+ "stations": "Korlevic Vision,Stuart Landing"
+ },
+ {
+ "name": "HIP 81996",
+ "pos_x": -89.25,
+ "pos_y": 98.8125,
+ "pos_z": 95.25,
+ "stations": "Einstein Camp,Carson Platform"
+ },
+ {
+ "name": "HIP 81998",
+ "pos_x": -65.03125,
+ "pos_y": 74.25,
+ "pos_z": 77.34375,
+ "stations": "Napier Ring"
+ },
+ {
+ "name": "HIP 82030",
+ "pos_x": -84.71875,
+ "pos_y": 81.96875,
+ "pos_z": 49.75,
+ "stations": "Ohm Colony"
+ },
+ {
+ "name": "HIP 82032",
+ "pos_x": 110.875,
+ "pos_y": -52.53125,
+ "pos_z": 128.71875,
+ "stations": "Stevens Point,Giles Colony"
+ },
+ {
+ "name": "HIP 82042",
+ "pos_x": -77.3125,
+ "pos_y": 76.8125,
+ "pos_z": 52.71875,
+ "stations": "Blodgett Platform"
+ },
+ {
+ "name": "HIP 82059",
+ "pos_x": -77.90625,
+ "pos_y": 90,
+ "pos_z": 98.28125,
+ "stations": "George Survey,Collins Base,Porges Laboratory"
+ },
+ {
+ "name": "HIP 82060",
+ "pos_x": -118.46875,
+ "pos_y": 121.9375,
+ "pos_z": 96.375,
+ "stations": "Vasilyev Beacon"
+ },
+ {
+ "name": "HIP 82068",
+ "pos_x": -125.375,
+ "pos_y": 122.3125,
+ "pos_z": 78.28125,
+ "stations": "Potter Vision"
+ },
+ {
+ "name": "HIP 82088",
+ "pos_x": 37.53125,
+ "pos_y": 17.71875,
+ "pos_z": 171.03125,
+ "stations": "Kotov Orbital,Altman Stop,Gibson Base"
+ },
+ {
+ "name": "HIP 8220",
+ "pos_x": -12.53125,
+ "pos_y": -130.6875,
+ "pos_z": -48.28125,
+ "stations": "Forest Settlement"
+ },
+ {
+ "name": "HIP 82206",
+ "pos_x": -111.25,
+ "pos_y": 93.1875,
+ "pos_z": 18.21875,
+ "stations": "Dunn Horizons,Kononenko Terminal,Hugh Base"
+ },
+ {
+ "name": "HIP 82233",
+ "pos_x": -8.34375,
+ "pos_y": 41.6875,
+ "pos_z": 130.6875,
+ "stations": "Tem Vision,Wilkes Legacy"
+ },
+ {
+ "name": "HIP 82303",
+ "pos_x": -31.625,
+ "pos_y": 72.625,
+ "pos_z": 181.34375,
+ "stations": "Antonio de Andrade Holdings"
+ },
+ {
+ "name": "HIP 82495",
+ "pos_x": -97.78125,
+ "pos_y": 81.03125,
+ "pos_z": 19.375,
+ "stations": "Townshend Terminal,Hirase Gateway,Detmer Dock"
+ },
+ {
+ "name": "HIP 8251",
+ "pos_x": 97.96875,
+ "pos_y": -237.78125,
+ "pos_z": 11.84375,
+ "stations": "Montanari Station,Schiltberger's Folly,Cassini Dock,Theodor Winnecke Hub,MacCready Plant"
+ },
+ {
+ "name": "HIP 82515",
+ "pos_x": 65.3125,
+ "pos_y": -11.09375,
+ "pos_z": 160.8125,
+ "stations": "Schouten Dock,Turzillo Horizons"
+ },
+ {
+ "name": "HIP 82521",
+ "pos_x": 31.09375,
+ "pos_y": 12.09375,
+ "pos_z": 147.1875,
+ "stations": "Cori Orbital"
+ },
+ {
+ "name": "HIP 82522",
+ "pos_x": -126.78125,
+ "pos_y": 91.90625,
+ "pos_z": -27.3125,
+ "stations": "Loncke Horizons,Chaviano Terminal,Dukaj Colony,Smith Penal colony"
+ },
+ {
+ "name": "HIP 82629",
+ "pos_x": -60.84375,
+ "pos_y": 92.53125,
+ "pos_z": 190.6875,
+ "stations": "Snowdon Port"
+ },
+ {
+ "name": "HIP 82636",
+ "pos_x": -112.59375,
+ "pos_y": 98.15625,
+ "pos_z": 46.75,
+ "stations": "Russ Installation,Steakley Plant,MacDonald's Progress"
+ },
+ {
+ "name": "HIP 82738",
+ "pos_x": 27.3125,
+ "pos_y": 21.0625,
+ "pos_z": 181.21875,
+ "stations": "Balmer Settlement,Alvarado Platform,Dorsey Beacon"
+ },
+ {
+ "name": "HIP 82820",
+ "pos_x": 72.25,
+ "pos_y": -31.59375,
+ "pos_z": 104.375,
+ "stations": "Werner von Siemens Port,Polyakov Station,Conrad Enterprise,Curbeam Station,Pawelczyk Enterprise,Oleskiw Station"
+ },
+ {
+ "name": "HIP 82835",
+ "pos_x": -33.28125,
+ "pos_y": 64.8125,
+ "pos_z": 173.40625,
+ "stations": "Simmons Dock,Janszoon Port,Watts Penal colony,Ledyard Depot"
+ },
+ {
+ "name": "HIP 82844",
+ "pos_x": -101.0625,
+ "pos_y": 84,
+ "pos_z": 29.625,
+ "stations": "Reed Lab,Davis Relay,Aikin Installation,Shatner Landing"
+ },
+ {
+ "name": "HIP 8285",
+ "pos_x": -92,
+ "pos_y": -97.5625,
+ "pos_z": -105.34375,
+ "stations": "Szilard Terminal"
+ },
+ {
+ "name": "HIP 82851",
+ "pos_x": 67.09375,
+ "pos_y": -27.4375,
+ "pos_z": 105.84375,
+ "stations": "McNair Dock,Arkwright Terminal,Behring Terminal,Hawley Terminal,Bursch Ring,Tyurin Port,Swanwick Survey,Murphy Works,Jensen Enterprise,Pettit Hub,Tuan Holdings,Monge Vision,Barnes Base,Kuhn Hub"
+ },
+ {
+ "name": "HIP 82886",
+ "pos_x": -128.84375,
+ "pos_y": 89.90625,
+ "pos_z": -37.6875,
+ "stations": "Plexico Plant,Vancouver Keep"
+ },
+ {
+ "name": "HIP 82896",
+ "pos_x": -134.375,
+ "pos_y": 97.71875,
+ "pos_z": -21.375,
+ "stations": "Lyell Dock,Haisheng Platform,Kagan Landing"
+ },
+ {
+ "name": "HIP 82954",
+ "pos_x": 81.71875,
+ "pos_y": -35.28125,
+ "pos_z": 122.46875,
+ "stations": "Elwood Station,Hill Enterprise,Nordenskiold Bastion,Gabriel Landing"
+ },
+ {
+ "name": "HIP 82964",
+ "pos_x": -137.3125,
+ "pos_y": 97.375,
+ "pos_z": -31.8125,
+ "stations": "Kessel Station,Drake Vision,Bryant's Inheritance,Veblen Terminal,Hoffman Mines"
+ },
+ {
+ "name": "HIP 83083",
+ "pos_x": -82.28125,
+ "pos_y": 86.875,
+ "pos_z": 117.09375,
+ "stations": "North Hub,Darlton Port,Levi-Civita Landing"
+ },
+ {
+ "name": "HIP 83099",
+ "pos_x": 3.59375,
+ "pos_y": 32.5625,
+ "pos_z": 168.03125,
+ "stations": "Nehsi Dock"
+ },
+ {
+ "name": "HIP 83137",
+ "pos_x": -59.21875,
+ "pos_y": 82.40625,
+ "pos_z": 182.3125,
+ "stations": "Lambert Base,Fidalgo Vision"
+ },
+ {
+ "name": "HIP 83163",
+ "pos_x": -10.0625,
+ "pos_y": 41.90625,
+ "pos_z": 167.1875,
+ "stations": "Popper Horizons,Griffith Orbital,Slonczewski Colony"
+ },
+ {
+ "name": "HIP 83181",
+ "pos_x": -30.09375,
+ "pos_y": 49.6875,
+ "pos_z": 132.9375,
+ "stations": "Grant Beacon,Jones Platform"
+ },
+ {
+ "name": "HIP 83195",
+ "pos_x": 8.6875,
+ "pos_y": 21.15625,
+ "pos_z": 136.09375,
+ "stations": "Hassanein Horizons,Watts Survey,Leiber Mines,Hoshi Platform"
+ },
+ {
+ "name": "HIP 83204",
+ "pos_x": -61.1875,
+ "pos_y": 67,
+ "pos_z": 104.28125,
+ "stations": "Ciferri Ring,Tarelkin Orbital,Leinster Penal colony,Erikson Installation"
+ },
+ {
+ "name": "HIP 83217",
+ "pos_x": 115.8125,
+ "pos_y": -69.9375,
+ "pos_z": 83.4375,
+ "stations": "Bainbridge Vision,Shen Enterprise"
+ },
+ {
+ "name": "HIP 83222",
+ "pos_x": -110.65625,
+ "pos_y": 90.40625,
+ "pos_z": 37.46875,
+ "stations": "Sverdrup Station,Diophantus Settlement"
+ },
+ {
+ "name": "HIP 83239",
+ "pos_x": 11.0625,
+ "pos_y": 21.5,
+ "pos_z": 148.53125,
+ "stations": "Hale Settlement,Jones Base"
+ },
+ {
+ "name": "HIP 83247",
+ "pos_x": 117.125,
+ "pos_y": -63.3125,
+ "pos_z": 121.71875,
+ "stations": "McDermott Arsenal,Abetti Dock,Delsanti Beacon"
+ },
+ {
+ "name": "HIP 83415",
+ "pos_x": 38.28125,
+ "pos_y": -3.5,
+ "pos_z": 131.78125,
+ "stations": "Soto Beacon,Moskowitz's Progress"
+ },
+ {
+ "name": "HIP 83425",
+ "pos_x": -92.40625,
+ "pos_y": 90.625,
+ "pos_z": 118.15625,
+ "stations": "Nicholson Station,Compton Gateway,Ashton Vision,Ivens Survey,Hannu Holdings"
+ },
+ {
+ "name": "HIP 83506",
+ "pos_x": 125.46875,
+ "pos_y": -36.8125,
+ "pos_z": 303.46875,
+ "stations": "Harvard Base"
+ },
+ {
+ "name": "HIP 83654",
+ "pos_x": -114.125,
+ "pos_y": 84.1875,
+ "pos_z": 4.5,
+ "stations": "Galois Arsenal,Tarski Base,Piserchia Laboratory"
+ },
+ {
+ "name": "HIP 83664",
+ "pos_x": -133.5625,
+ "pos_y": 90.5,
+ "pos_z": -40.8125,
+ "stations": "Fancher Arsenal,Burton Beacon"
+ },
+ {
+ "name": "HIP 83716",
+ "pos_x": -62.90625,
+ "pos_y": 73.46875,
+ "pos_z": 162.3125,
+ "stations": "Brackett Orbital,Ahern Vision,Kirtley Dock,Vardeman Relay"
+ },
+ {
+ "name": "HIP 83747",
+ "pos_x": -62.5625,
+ "pos_y": 66.03125,
+ "pos_z": 121.90625,
+ "stations": "Eisele Hub,Vonarburg's Folly"
+ },
+ {
+ "name": "HIP 83751",
+ "pos_x": 149.625,
+ "pos_y": -88.96875,
+ "pos_z": 118.21875,
+ "stations": "Schumacher Port,Nicholson Base,Bruce Prospect"
+ },
+ {
+ "name": "HIP 83874",
+ "pos_x": 27.75,
+ "pos_y": 4.25,
+ "pos_z": 151.53125,
+ "stations": "Coppel Terminal,Steiner Mines,Clark Orbital"
+ },
+ {
+ "name": "HIP 8389",
+ "pos_x": 56.53125,
+ "pos_y": -209.0625,
+ "pos_z": -16.15625,
+ "stations": "Farghani Hub,Bolton Vision,Card Terminal,Hartog's Progress,Norman's Inheritance"
+ },
+ {
+ "name": "HIP 83929",
+ "pos_x": -108.84375,
+ "pos_y": 85.875,
+ "pos_z": 48.34375,
+ "stations": "Temple Survey"
+ },
+ {
+ "name": "HIP 8396",
+ "pos_x": -226.5,
+ "pos_y": -12.46875,
+ "pos_z": -193.5,
+ "stations": "Stepping Stone Base,Titus Station,Foster's Beacon,Shackleton's Hope"
+ },
+ {
+ "name": "HIP 83983",
+ "pos_x": 92.15625,
+ "pos_y": -37.6875,
+ "pos_z": 186.28125,
+ "stations": "Wagner Works,Rubruck Terminal"
+ },
+ {
+ "name": "HIP 83995",
+ "pos_x": -112.28125,
+ "pos_y": 87.9375,
+ "pos_z": 49.1875,
+ "stations": "Cassidy Landing,Melvin Colony,Apt Orbital,Lavrador's Pride"
+ },
+ {
+ "name": "HIP 84164",
+ "pos_x": 54.96875,
+ "pos_y": -15.09375,
+ "pos_z": 169.375,
+ "stations": "Kirtley Vision,Laird Oasis,Cowper's Inheritance"
+ },
+ {
+ "name": "HIP 84169",
+ "pos_x": -142.09375,
+ "pos_y": 108.5625,
+ "pos_z": 54.71875,
+ "stations": "Stafford Estate,Stokes Camp,Schiltberger's Progress"
+ },
+ {
+ "name": "HIP 84182",
+ "pos_x": -28.15625,
+ "pos_y": 38.4375,
+ "pos_z": 131.53125,
+ "stations": "Weston Dock,Noli Prospect"
+ },
+ {
+ "name": "HIP 84253",
+ "pos_x": -48.59375,
+ "pos_y": 51.5,
+ "pos_z": 125.8125,
+ "stations": "Dyomin Legacy"
+ },
+ {
+ "name": "HIP 84255",
+ "pos_x": 51.09375,
+ "pos_y": -19.9375,
+ "pos_z": 118.875,
+ "stations": "Land Port,LeConte Hub,Zahn Enterprise"
+ },
+ {
+ "name": "HIP 84315",
+ "pos_x": 77.03125,
+ "pos_y": -43.15625,
+ "pos_z": 83.78125,
+ "stations": "Laumer Installation,Young Landing,Dilworth Terminal"
+ },
+ {
+ "name": "HIP 8438",
+ "pos_x": -4.34375,
+ "pos_y": -161.46875,
+ "pos_z": -53.21875,
+ "stations": "Westphal Reach,Bohme Survey,Hale Prospect"
+ },
+ {
+ "name": "HIP 8444",
+ "pos_x": 29.6875,
+ "pos_y": -241.46875,
+ "pos_z": -49.3125,
+ "stations": "Bliss Base"
+ },
+ {
+ "name": "HIP 8449",
+ "pos_x": 114.3125,
+ "pos_y": -232.59375,
+ "pos_z": 24.34375,
+ "stations": "Ikeya Vision"
+ },
+ {
+ "name": "HIP 84520",
+ "pos_x": -104.875,
+ "pos_y": 75.8125,
+ "pos_z": 23.4375,
+ "stations": "Bloch Refinery"
+ },
+ {
+ "name": "HIP 84633",
+ "pos_x": -48.8125,
+ "pos_y": 48.21875,
+ "pos_z": 127.75,
+ "stations": "Liebig Depot,Turtledove Penal colony"
+ },
+ {
+ "name": "HIP 84642",
+ "pos_x": 90.96875,
+ "pos_y": -44.5,
+ "pos_z": 163.34375,
+ "stations": "Aikin Camp,Cobb Vision"
+ },
+ {
+ "name": "HIP 84696",
+ "pos_x": -41.875,
+ "pos_y": 41.75,
+ "pos_z": 117.53125,
+ "stations": "Sinclair Dock"
+ },
+ {
+ "name": "HIP 84787",
+ "pos_x": 57.40625,
+ "pos_y": -21.84375,
+ "pos_z": 167.8125,
+ "stations": "Phillpotts Holdings"
+ },
+ {
+ "name": "HIP 84816",
+ "pos_x": -113.34375,
+ "pos_y": 79.46875,
+ "pos_z": 17.25,
+ "stations": "Kingsmill Prospect,Wilder Refinery,Maddox Beacon"
+ },
+ {
+ "name": "HIP 84838",
+ "pos_x": -96.0625,
+ "pos_y": 74.875,
+ "pos_z": 89.375,
+ "stations": "McKay Colony"
+ },
+ {
+ "name": "HIP 8489",
+ "pos_x": -74.03125,
+ "pos_y": -111.875,
+ "pos_z": -97.09375,
+ "stations": "Ziemianski Base,Payne Port"
+ },
+ {
+ "name": "HIP 84913",
+ "pos_x": -38.28125,
+ "pos_y": 44.25,
+ "pos_z": 184.75,
+ "stations": "Bacigalupi Terminal,Bachman Stop,Montrose Keep"
+ },
+ {
+ "name": "HIP 85010",
+ "pos_x": 54.4375,
+ "pos_y": -25.375,
+ "pos_z": 125.21875,
+ "stations": "Rominger Platform,Weyn Installation,Volynov Platform"
+ },
+ {
+ "name": "HIP 85038",
+ "pos_x": 90.15625,
+ "pos_y": -45.0625,
+ "pos_z": 176.3125,
+ "stations": "Terry's Inheritance"
+ },
+ {
+ "name": "HIP 85064",
+ "pos_x": -56.34375,
+ "pos_y": 55.28125,
+ "pos_z": 192.59375,
+ "stations": "Dall Dock,Alvarez de Pineda Terminal,King Lab,Anderson Depot"
+ },
+ {
+ "name": "HIP 8507",
+ "pos_x": 4.46875,
+ "pos_y": -177.78125,
+ "pos_z": -51.8125,
+ "stations": "Al-Khujandi Landing,Hevelius Dock"
+ },
+ {
+ "name": "HIP 85129",
+ "pos_x": -70.21875,
+ "pos_y": 55.71875,
+ "pos_z": 98.65625,
+ "stations": "Aleksandrov Holdings,Paulmier de Gonneville Hub,Herreshoff Hub,Cavendish Terminal,Hayden Landing,Cavalieri Arsenal"
+ },
+ {
+ "name": "HIP 85141",
+ "pos_x": -46.3125,
+ "pos_y": 46.46875,
+ "pos_z": 179.90625,
+ "stations": "Pordenone Dock,Alexander Barracks,Cook Enterprise"
+ },
+ {
+ "name": "HIP 85244",
+ "pos_x": -110.9375,
+ "pos_y": 75.75,
+ "pos_z": 18.53125,
+ "stations": "Walker Orbital,Quick Station"
+ },
+ {
+ "name": "HIP 8525",
+ "pos_x": -96.28125,
+ "pos_y": 31.65625,
+ "pos_z": -71.25,
+ "stations": "Fabian Beacon,Wiberg Platform,Wohler Mines,Antonio de Andrade Beacon,Buckland Mines"
+ },
+ {
+ "name": "HIP 8527",
+ "pos_x": 61.0625,
+ "pos_y": -239.25,
+ "pos_z": -23.59375,
+ "stations": "Zenbei Prospect,McQuarrie Terminal,Herbig Prospect"
+ },
+ {
+ "name": "HIP 85285",
+ "pos_x": -10.9375,
+ "pos_y": 19.0625,
+ "pos_z": 152.1875,
+ "stations": "Samuda Dock,Dashiell Settlement"
+ },
+ {
+ "name": "HIP 85297",
+ "pos_x": 105.5625,
+ "pos_y": -61.65625,
+ "pos_z": 115.90625,
+ "stations": "Hausdorff Landing,Giraud Gateway,Carver's Progress,Coggia Hub,Rutan Orbital"
+ },
+ {
+ "name": "HIP 85360",
+ "pos_x": 11.3125,
+ "pos_y": 1.4375,
+ "pos_z": 123.1875,
+ "stations": "Macan Platform"
+ },
+ {
+ "name": "HIP 85414",
+ "pos_x": -125.84375,
+ "pos_y": 87.09375,
+ "pos_z": 52,
+ "stations": "Still Gateway,Ryman Installation"
+ },
+ {
+ "name": "HIP 85437",
+ "pos_x": -114.375,
+ "pos_y": 82.1875,
+ "pos_z": 94.78125,
+ "stations": "Bowersox Vision,Klink's Folly"
+ },
+ {
+ "name": "HIP 8548",
+ "pos_x": -19.75,
+ "pos_y": -127.5,
+ "pos_z": -56.9375,
+ "stations": "Harawi Terminal,Lassell Holdings"
+ },
+ {
+ "name": "HIP 85533",
+ "pos_x": 25.5,
+ "pos_y": -9.46875,
+ "pos_z": 117.5,
+ "stations": "Weston Refinery,Ivins' Progress,Akiyama Refinery"
+ },
+ {
+ "name": "HIP 85575",
+ "pos_x": -123.28125,
+ "pos_y": 80.5625,
+ "pos_z": -7.28125,
+ "stations": "Lopez de Legazpi Port,Stevens Enterprise"
+ },
+ {
+ "name": "HIP 85639",
+ "pos_x": -101.0625,
+ "pos_y": 68.1875,
+ "pos_z": 36.125,
+ "stations": "Shelley City,Schiltberger Enterprise,Starzl Ring,Key Settlement,Griffith Hub"
+ },
+ {
+ "name": "HIP 8564",
+ "pos_x": -12.15625,
+ "pos_y": -179.03125,
+ "pos_z": -67.03125,
+ "stations": "Abetti Vision"
+ },
+ {
+ "name": "HIP 85686",
+ "pos_x": -106.59375,
+ "pos_y": 71.40625,
+ "pos_z": 33.625,
+ "stations": "Tokubei Relay,Murphy Point,Normand Terminal"
+ },
+ {
+ "name": "HIP 85704",
+ "pos_x": -76.40625,
+ "pos_y": 58.4375,
+ "pos_z": 162.5,
+ "stations": "Lark's Rise"
+ },
+ {
+ "name": "HIP 8582",
+ "pos_x": -109.3125,
+ "pos_y": -14.46875,
+ "pos_z": -97.125,
+ "stations": "Langford Terminal,Griffiths Holdings,Linaweaver Prospect,Andrews Hub"
+ },
+ {
+ "name": "HIP 85850",
+ "pos_x": -111.0625,
+ "pos_y": 74.9375,
+ "pos_z": 69.625,
+ "stations": "Curie Outpost,Stewart Beacon"
+ },
+ {
+ "name": "HIP 85887",
+ "pos_x": -110.34375,
+ "pos_y": 69.90625,
+ "pos_z": -31.375,
+ "stations": "Marshburn Terminal,Rushd Terminal,Blodgett Orbital,Low Terminal,Howe Orbital,Lanchester Dock,Thagard Orbital,Laird Point"
+ },
+ {
+ "name": "HIP 8593",
+ "pos_x": 107.46875,
+ "pos_y": -226.8125,
+ "pos_z": 18.53125,
+ "stations": "Elson Landing,Carroll Oasis,Chadwick Barracks"
+ },
+ {
+ "name": "HIP 85960",
+ "pos_x": 53.5625,
+ "pos_y": -30.53125,
+ "pos_z": 103.0625,
+ "stations": "Fermat Dock,Iqbal Dock"
+ },
+ {
+ "name": "HIP 86063",
+ "pos_x": -127.84375,
+ "pos_y": 86.1875,
+ "pos_z": 139.625,
+ "stations": "Chasles Landing,Velho Platform,Killough Vision,Benyovszky Dock"
+ },
+ {
+ "name": "HIP 86173",
+ "pos_x": -145,
+ "pos_y": 93.125,
+ "pos_z": 38.5625,
+ "stations": "Herzfeld Platform,Mohmand's Folly,Chiao Camp"
+ },
+ {
+ "name": "HIP 862",
+ "pos_x": 55.84375,
+ "pos_y": -200.03125,
+ "pos_z": 64.4375,
+ "stations": "Kanai Terminal,Hay Ring,Fabricius Hub,Carpenter Keep,Wood Camp"
+ },
+ {
+ "name": "HIP 8623",
+ "pos_x": 166.0625,
+ "pos_y": -234.40625,
+ "pos_z": 65.46875,
+ "stations": "Peltier Outpost,Bruce's Claim,Cowell Port"
+ },
+ {
+ "name": "HIP 86385",
+ "pos_x": -3.78125,
+ "pos_y": 4.875,
+ "pos_z": 167.5625,
+ "stations": "Ziemianski Enterprise,Ford Platform,Collins Depot"
+ },
+ {
+ "name": "HIP 86419",
+ "pos_x": 19.96875,
+ "pos_y": -10.90625,
+ "pos_z": 123.40625,
+ "stations": "Laing Freeport"
+ },
+ {
+ "name": "HIP 86431",
+ "pos_x": -139.46875,
+ "pos_y": 88.25,
+ "pos_z": 73.09375,
+ "stations": "Makeev Installation,McHugh Works"
+ },
+ {
+ "name": "HIP 86470",
+ "pos_x": -75.21875,
+ "pos_y": 48.625,
+ "pos_z": 151.1875,
+ "stations": "Merril Prospect,Bridger Terminal,Heck Horizons"
+ },
+ {
+ "name": "HIP 8653",
+ "pos_x": -18.34375,
+ "pos_y": -135.84375,
+ "pos_z": -59.46875,
+ "stations": "Dana Terminal,Swift City,Bordage Vision,Perga Base"
+ },
+ {
+ "name": "HIP 86568",
+ "pos_x": -159.65625,
+ "pos_y": 98.96875,
+ "pos_z": -31.0625,
+ "stations": "Huxley Hub,Rasch City,Okorafor Enterprise,Grant's Progress"
+ },
+ {
+ "name": "HIP 86644",
+ "pos_x": -62.40625,
+ "pos_y": 38.6875,
+ "pos_z": 109.46875,
+ "stations": "Forbes Ring,Bursch Barracks"
+ },
+ {
+ "name": "HIP 86691",
+ "pos_x": 65.625,
+ "pos_y": -40.75,
+ "pos_z": 135.59375,
+ "stations": "Issigonis Outpost,Gardner Settlement"
+ },
+ {
+ "name": "HIP 8674",
+ "pos_x": -1.09375,
+ "pos_y": -162.78125,
+ "pos_z": -53.6875,
+ "stations": "Wilhelm von Struve Orbital,Jeschke Bastion"
+ },
+ {
+ "name": "HIP 86775",
+ "pos_x": -170.90625,
+ "pos_y": 105.0625,
+ "pos_z": -28.1875,
+ "stations": "Lewis Base"
+ },
+ {
+ "name": "HIP 86882",
+ "pos_x": -99.28125,
+ "pos_y": 59.375,
+ "pos_z": 103.375,
+ "stations": "Bella Vision"
+ },
+ {
+ "name": "HIP 86950",
+ "pos_x": -108.28125,
+ "pos_y": 64.625,
+ "pos_z": 70.0625,
+ "stations": "Tavernier Settlement"
+ },
+ {
+ "name": "HIP 870",
+ "pos_x": -91.3125,
+ "pos_y": -157.46875,
+ "pos_z": -22.90625,
+ "stations": "MacDonald Prospect"
+ },
+ {
+ "name": "HIP 87034",
+ "pos_x": -58.65625,
+ "pos_y": 32.25,
+ "pos_z": 159.75,
+ "stations": "Belyanin Vision,Chios Colony,Nelson Settlement"
+ },
+ {
+ "name": "HIP 87100",
+ "pos_x": 78.3125,
+ "pos_y": -50.4375,
+ "pos_z": 134.75,
+ "stations": "Gold Prospect,Apianus Landing"
+ },
+ {
+ "name": "HIP 8711",
+ "pos_x": 148.75,
+ "pos_y": -261.53125,
+ "pos_z": 41.1875,
+ "stations": "Bolkow Hub,Folland Horizons,Tall's Inheritance"
+ },
+ {
+ "name": "HIP 87135",
+ "pos_x": -134.21875,
+ "pos_y": 79,
+ "pos_z": 65.5625,
+ "stations": "Ozanne Terminal"
+ },
+ {
+ "name": "HIP 87154",
+ "pos_x": 79.53125,
+ "pos_y": -51.875,
+ "pos_z": 153.375,
+ "stations": "Joy Point,Arzachel City"
+ },
+ {
+ "name": "HIP 87204",
+ "pos_x": -132.84375,
+ "pos_y": 77.5625,
+ "pos_z": 68.46875,
+ "stations": "Friend Enterprise,Sharman Dock,Curie Terminal"
+ },
+ {
+ "name": "HIP 8722",
+ "pos_x": 81.4375,
+ "pos_y": -255.1875,
+ "pos_z": -14.15625,
+ "stations": "Fraunhofer Colony,Marius Colony"
+ },
+ {
+ "name": "HIP 87369",
+ "pos_x": -5.625,
+ "pos_y": -2.09375,
+ "pos_z": 140.3125,
+ "stations": "Carey Port,Kerwin Orbital,Landsteiner Gateway"
+ },
+ {
+ "name": "HIP 87370",
+ "pos_x": -14,
+ "pos_y": 3.25,
+ "pos_z": 129.96875,
+ "stations": "Lamarck Retreat"
+ },
+ {
+ "name": "HIP 87414",
+ "pos_x": 164.3125,
+ "pos_y": -102.75,
+ "pos_z": 134.6875,
+ "stations": "Blaauw City,Harris Terminal,Neujmin Depot,Schaumasse Port,Bonestell Vision"
+ },
+ {
+ "name": "HIP 8744",
+ "pos_x": 99.34375,
+ "pos_y": -163.0625,
+ "pos_z": 31.0625,
+ "stations": "Goodman Stop"
+ },
+ {
+ "name": "HIP 8753",
+ "pos_x": -92.625,
+ "pos_y": -62,
+ "pos_z": -99.4375,
+ "stations": "Jernigan Landing,Fuglesang Vision"
+ },
+ {
+ "name": "HIP 8758",
+ "pos_x": 35.125,
+ "pos_y": -236.9375,
+ "pos_z": -48.28125,
+ "stations": "Firsoff Orbital"
+ },
+ {
+ "name": "HIP 87594",
+ "pos_x": -140.15625,
+ "pos_y": 82.09375,
+ "pos_z": 0,
+ "stations": "Culbertson Platform"
+ },
+ {
+ "name": "HIP 87638",
+ "pos_x": 107.15625,
+ "pos_y": -68.9375,
+ "pos_z": 121.5625,
+ "stations": "Moskowitz Barracks,Libeskind Survey,Humason Dock,Holden Terminal"
+ },
+ {
+ "name": "HIP 87658",
+ "pos_x": 22.84375,
+ "pos_y": -20.34375,
+ "pos_z": 133.46875,
+ "stations": "Bounds Installation,Stapledon Ring,Wells Gateway,Williams Orbital,Corte-Real Terminal,Bertin Terminal,Gilliland City,Clairaut Orbital,Yolen Enterprise"
+ },
+ {
+ "name": "HIP 87678",
+ "pos_x": -66.9375,
+ "pos_y": 30.8125,
+ "pos_z": 153.65625,
+ "stations": "Temple Installation"
+ },
+ {
+ "name": "HIP 87679",
+ "pos_x": -9.03125,
+ "pos_y": -3.03125,
+ "pos_z": 154.9375,
+ "stations": "Qureshi Dock,Hughes-Fulford Orbital"
+ },
+ {
+ "name": "HIP 87710",
+ "pos_x": -47.9375,
+ "pos_y": 20.53125,
+ "pos_z": 133.125,
+ "stations": "Shepard Platform,Fraley Settlement,Block Base,Piserchia Settlement"
+ },
+ {
+ "name": "HIP 8775",
+ "pos_x": -41.21875,
+ "pos_y": -118.125,
+ "pos_z": -74.3125,
+ "stations": "Otiman Camp,Hardwick Camp"
+ },
+ {
+ "name": "HIP 87800",
+ "pos_x": -105.71875,
+ "pos_y": 55.21875,
+ "pos_z": 98.21875,
+ "stations": "Thorne Terminal,Hutton Landing,Conti Hub"
+ },
+ {
+ "name": "HIP 87914",
+ "pos_x": 50.875,
+ "pos_y": -36.84375,
+ "pos_z": 113.96875,
+ "stations": "Hobaugh Hub,Drebbel Dock,Ramelli Terminal,Shriver Orbital,Wang Station,Flade Hub,Farkas Hub,Olivas Orbital,Musabayev Orbital,Kratman Forum,Alvares Vision,Newton's Progress"
+ },
+ {
+ "name": "HIP 8807",
+ "pos_x": 55.8125,
+ "pos_y": -247.25,
+ "pos_z": -34.625,
+ "stations": "Smith Retreat,Trujillo Mine"
+ },
+ {
+ "name": "HIP 88166",
+ "pos_x": -99.34375,
+ "pos_y": 47.25,
+ "pos_z": 114.5,
+ "stations": "Parker Enterprise,Usachov Port,Slayton Landing,Qian Silo"
+ },
+ {
+ "name": "HIP 88178",
+ "pos_x": 53.125,
+ "pos_y": -41.25,
+ "pos_z": 140.0625,
+ "stations": "Volta Port,Stephenson Bastion,McKee Camp,Illy Platform,Griggs Dock"
+ },
+ {
+ "name": "HIP 88227",
+ "pos_x": -91.125,
+ "pos_y": 41.78125,
+ "pos_z": 118.4375,
+ "stations": "Nolan Landing,Tanaka Horizons,Crown Orbital"
+ },
+ {
+ "name": "HIP 8825",
+ "pos_x": -111.21875,
+ "pos_y": 29.59375,
+ "pos_z": -85.3125,
+ "stations": "Lyakhov City,Haber City,Ross Terminal,Oliver Installation"
+ },
+ {
+ "name": "HIP 8830",
+ "pos_x": 8.40625,
+ "pos_y": -123.6875,
+ "pos_z": -34.25,
+ "stations": "Gould Ring,Marius Station,Miyasaka Port,Perrine Station,Cassini City,Abel Port,Riazuddin Station,Smoot Prospect,Eskridge Base,Young Refinery,Lindblad Station,Hoffmeister Port,Hanke-Woods Terminal,Whipple Vista"
+ },
+ {
+ "name": "HIP 88353",
+ "pos_x": -65.625,
+ "pos_y": 27.375,
+ "pos_z": 106.65625,
+ "stations": "Kennan Camp,Caryanda Arsenal,Russ Camp"
+ },
+ {
+ "name": "HIP 88399",
+ "pos_x": 47.15625,
+ "pos_y": -39.46875,
+ "pos_z": 144.46875,
+ "stations": "Potrykus Mine,Malenchenko Outpost,White's Folly"
+ },
+ {
+ "name": "HIP 88471",
+ "pos_x": -117.59375,
+ "pos_y": 58.21875,
+ "pos_z": 77.21875,
+ "stations": "Nelder Station,Sekelj Enterprise,Lefschetz Beacon,Klein Dock"
+ },
+ {
+ "name": "HIP 88553",
+ "pos_x": 25.28125,
+ "pos_y": -26.21875,
+ "pos_z": 123.96875,
+ "stations": "McIntyre Works,Zettel Platform"
+ },
+ {
+ "name": "HIP 88572",
+ "pos_x": -147.4375,
+ "pos_y": 79.40625,
+ "pos_z": 22.25,
+ "stations": "Garcia Port,Archer Station"
+ },
+ {
+ "name": "HIP 8859",
+ "pos_x": -47.5625,
+ "pos_y": -101.84375,
+ "pos_z": -75.21875,
+ "stations": "Baturin Orbital,Ivanov Enterprise,Torricelli Port,Apgar City,Ray Ring,Voss Port"
+ },
+ {
+ "name": "HIP 88595",
+ "pos_x": -28.28125,
+ "pos_y": -2.5625,
+ "pos_z": 181.125,
+ "stations": "Clark Orbital,Sauma Camp,Rond d'Alembert Lab"
+ },
+ {
+ "name": "HIP 88617",
+ "pos_x": -108.75,
+ "pos_y": 60.625,
+ "pos_z": -5.875,
+ "stations": "Cenker City,Stephenson Station"
+ },
+ {
+ "name": "HIP 88633",
+ "pos_x": 46.21875,
+ "pos_y": -43.1875,
+ "pos_z": 172.84375,
+ "stations": "Haber Beacon,Stabenow Terminal"
+ },
+ {
+ "name": "HIP 88644",
+ "pos_x": -84.9375,
+ "pos_y": 34.125,
+ "pos_z": 123.90625,
+ "stations": "Zelazny Hub,Schweinfurth Holdings"
+ },
+ {
+ "name": "HIP 8865",
+ "pos_x": -92.6875,
+ "pos_y": -4.34375,
+ "pos_z": -81.03125,
+ "stations": "Solovyev Port,Covey Enterprise,Phillips Survey,Barth Survey"
+ },
+ {
+ "name": "HIP 88656",
+ "pos_x": -63.78125,
+ "pos_y": 17.84375,
+ "pos_z": 167.78125,
+ "stations": "al-Haytham's Folly,Scithers Depot"
+ },
+ {
+ "name": "HIP 88726",
+ "pos_x": 23.75,
+ "pos_y": -27.09375,
+ "pos_z": 131.625,
+ "stations": "Abbott Beacon,Barba Orbital,Gooch Terminal,Carver City,Banks Dock,Preuss Beacon,Lundwall Colony,Spedding City,Sagan Dock,Anderson Terminal"
+ },
+ {
+ "name": "HIP 88728",
+ "pos_x": -75.71875,
+ "pos_y": 30.5,
+ "pos_z": 103.53125,
+ "stations": "Tito Ring,Swanson Horizons,Sohl Arena,Chamitoff Mines,Grassmann Installation"
+ },
+ {
+ "name": "HIP 88924",
+ "pos_x": -59.78125,
+ "pos_y": 13.3125,
+ "pos_z": 164.1875,
+ "stations": "Leckie Hub,Auld Vision,Porges Vision,Lerner Silo"
+ },
+ {
+ "name": "HIP 8894",
+ "pos_x": 44.59375,
+ "pos_y": -176.90625,
+ "pos_z": -21.96875,
+ "stations": "Shea City,Bullialdus Gateway,Lee Terminal,Trujillo's Progress"
+ },
+ {
+ "name": "HIP 89100",
+ "pos_x": -119.34375,
+ "pos_y": 55.34375,
+ "pos_z": 70,
+ "stations": "Erikson's Pride,Harrison Relay,Ahmed Landing"
+ },
+ {
+ "name": "HIP 89207",
+ "pos_x": -98.59375,
+ "pos_y": 30.84375,
+ "pos_z": 167.6875,
+ "stations": "Alexander Enterprise"
+ },
+ {
+ "name": "HIP 89285",
+ "pos_x": 77.28125,
+ "pos_y": -62.59375,
+ "pos_z": 161.3125,
+ "stations": "Safdie Dock,Brorsen Port,Napier's Folly,van Royen Station"
+ },
+ {
+ "name": "HIP 89294",
+ "pos_x": -129.15625,
+ "pos_y": 50.53125,
+ "pos_z": 134.84375,
+ "stations": "Gaspar de Lemos Vision"
+ },
+ {
+ "name": "HIP 89478",
+ "pos_x": -166.6875,
+ "pos_y": 96.46875,
+ "pos_z": -62.90625,
+ "stations": "Bond City,Naddoddur Penal colony,Sturgeon Horizons"
+ },
+ {
+ "name": "HIP 8972",
+ "pos_x": -32.625,
+ "pos_y": -107.40625,
+ "pos_z": -65.53125,
+ "stations": "Vonarburg Survey,Born Beacon"
+ },
+ {
+ "name": "HIP 898",
+ "pos_x": -71.90625,
+ "pos_y": -111.375,
+ "pos_z": -20.46875,
+ "stations": "Fadlan Hub,Lie Platform,Musgrave Keep"
+ },
+ {
+ "name": "HIP 89806",
+ "pos_x": -115.46875,
+ "pos_y": 36.28125,
+ "pos_z": 143.15625,
+ "stations": "Kimberlin Port"
+ },
+ {
+ "name": "HIP 89911",
+ "pos_x": -177.75,
+ "pos_y": 96.15625,
+ "pos_z": -32.875,
+ "stations": "Cavalieri Outpost"
+ },
+ {
+ "name": "HIP 90004",
+ "pos_x": -46.09375,
+ "pos_y": 1.0625,
+ "pos_z": 131.53125,
+ "stations": "Stirling Orbital,Heaviside Installation"
+ },
+ {
+ "name": "HIP 90055",
+ "pos_x": -32.5,
+ "pos_y": -6.125,
+ "pos_z": 130.03125,
+ "stations": "Barsanti City,Soper Barracks,Smith Observatory"
+ },
+ {
+ "name": "HIP 90112",
+ "pos_x": 14.65625,
+ "pos_y": -35.40625,
+ "pos_z": 159.09375,
+ "stations": "Kubasov Station,Piaget Orbital,Townshend Settlement,Guerrero Camp,McHugh Depot"
+ },
+ {
+ "name": "HIP 90151",
+ "pos_x": 25.15625,
+ "pos_y": -36.90625,
+ "pos_z": 136.03125,
+ "stations": "Dobrovolskiy Colony,Zahn Arsenal,Garn Landing"
+ },
+ {
+ "name": "HIP 90162",
+ "pos_x": 50.40625,
+ "pos_y": -44.625,
+ "pos_z": 108.09375,
+ "stations": "Lopez de Villalobos Dock,Dorsey Ring,Williams Dock"
+ },
+ {
+ "name": "HIP 90190",
+ "pos_x": -132.71875,
+ "pos_y": 51.46875,
+ "pos_z": 83.6875,
+ "stations": "Bolger Port,Brooks Port,Smith Orbital,Baker Bastion"
+ },
+ {
+ "name": "HIP 90212",
+ "pos_x": -10.4375,
+ "pos_y": -22.03125,
+ "pos_z": 150.59375,
+ "stations": "Bayley Landing,Savorgnan de Brazza Enterprise"
+ },
+ {
+ "name": "HIP 90235",
+ "pos_x": -137.21875,
+ "pos_y": 54.875,
+ "pos_z": 75.59375,
+ "stations": "Edison Dock,Pettit Landing,Harris Laboratory,Cantor Laboratory"
+ },
+ {
+ "name": "HIP 90246",
+ "pos_x": -52.71875,
+ "pos_y": 5.3125,
+ "pos_z": 115.25,
+ "stations": "Payette Ring,Ramsay Gateway"
+ },
+ {
+ "name": "HIP 90415",
+ "pos_x": -137.71875,
+ "pos_y": 57.84375,
+ "pos_z": 53.1875,
+ "stations": "Ramelli Landing,Shriver Platform"
+ },
+ {
+ "name": "HIP 90451",
+ "pos_x": -26.90625,
+ "pos_y": -14.65625,
+ "pos_z": 144.5,
+ "stations": "Neff Enterprise,Clairaut Vision,Hawke Vision,Grzimek Camp"
+ },
+ {
+ "name": "HIP 90459",
+ "pos_x": -44.125,
+ "pos_y": -6.375,
+ "pos_z": 145.0625,
+ "stations": "Abernathy Terminal"
+ },
+ {
+ "name": "HIP 90467",
+ "pos_x": -96.75,
+ "pos_y": 18.3125,
+ "pos_z": 150.90625,
+ "stations": "Ashton Installation"
+ },
+ {
+ "name": "HIP 9051",
+ "pos_x": 151.75,
+ "pos_y": -242.09375,
+ "pos_z": 46.0625,
+ "stations": "Galle Colony,Bullialdus Port,Brom Beacon"
+ },
+ {
+ "name": "HIP 9052",
+ "pos_x": 164.75,
+ "pos_y": -135.6875,
+ "pos_z": 95,
+ "stations": "Jones Port,Celsius Terminal,Sudworth Terminal,Williamson Keep"
+ },
+ {
+ "name": "HIP 90539",
+ "pos_x": -56.84375,
+ "pos_y": 6,
+ "pos_z": 110.625,
+ "stations": "Diesel Refinery"
+ },
+ {
+ "name": "HIP 90578",
+ "pos_x": -81.75,
+ "pos_y": 7.78125,
+ "pos_z": 161.0625,
+ "stations": "Karlsefni Holdings"
+ },
+ {
+ "name": "HIP 9059",
+ "pos_x": -103.375,
+ "pos_y": -45.28125,
+ "pos_z": -105.78125,
+ "stations": "Smith Point"
+ },
+ {
+ "name": "HIP 90596",
+ "pos_x": -12.21875,
+ "pos_y": -24.03125,
+ "pos_z": 149.4375,
+ "stations": "Nusslein-Volhard Depot,Nakaya Dock"
+ },
+ {
+ "name": "HIP 90598",
+ "pos_x": 45.4375,
+ "pos_y": -47.75,
+ "pos_z": 127.4375,
+ "stations": "Ryan Station,Freas Settlement,Hussenot Vision"
+ },
+ {
+ "name": "HIP 90621",
+ "pos_x": -120.5,
+ "pos_y": 26.84375,
+ "pos_z": 157.9375,
+ "stations": "Aucharnie"
+ },
+ {
+ "name": "HIP 90708",
+ "pos_x": -181,
+ "pos_y": 69.625,
+ "pos_z": 87.84375,
+ "stations": "Starzl Prospect"
+ },
+ {
+ "name": "HIP 90753",
+ "pos_x": 56.875,
+ "pos_y": -59.75,
+ "pos_z": 155.03125,
+ "stations": "Anthony Orbital,Kurland Port,Bates Vision,Lalande Penal colony,Shaara's Folly"
+ },
+ {
+ "name": "HIP 90760",
+ "pos_x": -154.75,
+ "pos_y": 82.6875,
+ "pos_z": -38.375,
+ "stations": "Womack Camp"
+ },
+ {
+ "name": "HIP 90810",
+ "pos_x": -100.15625,
+ "pos_y": 11.09375,
+ "pos_z": 176.75,
+ "stations": "Chun Mines,Anthony de la Roche Works"
+ },
+ {
+ "name": "HIP 90848",
+ "pos_x": 25.59375,
+ "pos_y": -40.21875,
+ "pos_z": 131.59375,
+ "stations": "Conway Mine"
+ },
+ {
+ "name": "HIP 90954",
+ "pos_x": -119.0625,
+ "pos_y": 46.6875,
+ "pos_z": 46.34375,
+ "stations": "Mendeleev Relay,Borlaug Dock"
+ },
+ {
+ "name": "HIP 91043",
+ "pos_x": -90.59375,
+ "pos_y": 24.9375,
+ "pos_z": 81,
+ "stations": "McQuay Depot,Hobaugh Terminal,Musgrave Port"
+ },
+ {
+ "name": "HIP 91058",
+ "pos_x": -137.46875,
+ "pos_y": 49.5625,
+ "pos_z": 69.625,
+ "stations": "Carr Station,Duffy Station,Yurchikhin Port,Boodt Camp"
+ },
+ {
+ "name": "HIP 91085",
+ "pos_x": -17.75,
+ "pos_y": -27.53125,
+ "pos_z": 160.65625,
+ "stations": "Vinci Ring,Cremona Keep,Lem Camp"
+ },
+ {
+ "name": "HIP 91138",
+ "pos_x": -116.65625,
+ "pos_y": 28.8125,
+ "pos_z": 115.21875,
+ "stations": "Baxter Survey,Chern Survey"
+ },
+ {
+ "name": "HIP 91187",
+ "pos_x": -50.28125,
+ "pos_y": -5.15625,
+ "pos_z": 125.25,
+ "stations": "Thompson Port"
+ },
+ {
+ "name": "HIP 91229",
+ "pos_x": -154.5,
+ "pos_y": 74.3125,
+ "pos_z": -8.71875,
+ "stations": "Liebig Port,Williams Hub,DeLucas Lab"
+ },
+ {
+ "name": "HIP 91253",
+ "pos_x": -22.15625,
+ "pos_y": -25.65625,
+ "pos_z": 155.0625,
+ "stations": "Comino Platform,Gantt Port,Clifford Laboratory"
+ },
+ {
+ "name": "HIP 9127",
+ "pos_x": -17.9375,
+ "pos_y": -114.1875,
+ "pos_z": -56.78125,
+ "stations": "Everest Vision,Steele Colony"
+ },
+ {
+ "name": "HIP 91281",
+ "pos_x": -93.65625,
+ "pos_y": 9.3125,
+ "pos_z": 147.0625,
+ "stations": "Stapledon Dock,Benyovszky Station,Tokubei Enterprise,Person Keep,Besonders Beacon"
+ },
+ {
+ "name": "HIP 91295",
+ "pos_x": -108.34375,
+ "pos_y": 22.9375,
+ "pos_z": 117.625,
+ "stations": "Galouye Dock,Davis Port,Dorsey Lab,Fisk Base"
+ },
+ {
+ "name": "HIP 91337",
+ "pos_x": -78.125,
+ "pos_y": 0.8125,
+ "pos_z": 150.4375,
+ "stations": "Shkaplerov Port,Torricelli Base"
+ },
+ {
+ "name": "HIP 91371",
+ "pos_x": -151.9375,
+ "pos_y": 69.15625,
+ "pos_z": 5.5,
+ "stations": "Howard Point,Cochrane Installation,Low Terminal"
+ },
+ {
+ "name": "HIP 914",
+ "pos_x": 1.65625,
+ "pos_y": -196.84375,
+ "pos_z": 33.84375,
+ "stations": "Fan Terminal,Lowell Base,Cheranovsky Holdings"
+ },
+ {
+ "name": "HIP 91404",
+ "pos_x": 35.28125,
+ "pos_y": -43.90625,
+ "pos_z": 115.28125,
+ "stations": "Barjavel Vision,Helmholtz Beacon,Birdseye Platform"
+ },
+ {
+ "name": "HIP 9141",
+ "pos_x": 11.34375,
+ "pos_y": -127.875,
+ "pos_z": -36.28125,
+ "stations": "Jean Terminal,Brooks Vision,Trumpler Station,Karachkina Orbital,Waldeck Silo,Webb Landing,Hayashi Orbital,Farghani Gateway,Greene Ring,Perry's Inheritance"
+ },
+ {
+ "name": "HIP 91434",
+ "pos_x": 146.3125,
+ "pos_y": -94.9375,
+ "pos_z": 113.5625,
+ "stations": "Perrine Station,Mil Vision,Wolszczan Port,Williams Installation,Bierce Point"
+ },
+ {
+ "name": "HIP 9146",
+ "pos_x": 60.84375,
+ "pos_y": -247.09375,
+ "pos_z": -36.21875,
+ "stations": "Dilworth Relay"
+ },
+ {
+ "name": "HIP 91479",
+ "pos_x": -120.0625,
+ "pos_y": 36.84375,
+ "pos_z": 75.75,
+ "stations": "Singer Dock,Bogdanov Stop,Carver Mine"
+ },
+ {
+ "name": "HIP 91507",
+ "pos_x": -132.25,
+ "pos_y": 47.09375,
+ "pos_z": 56.03125,
+ "stations": "Lichtenberg Depot,Cousin Outpost"
+ },
+ {
+ "name": "HIP 91563",
+ "pos_x": -146.03125,
+ "pos_y": 48.40625,
+ "pos_z": 74.75,
+ "stations": "Grandin Station,Bramah Park,Mukai Port,Jenner Terminal"
+ },
+ {
+ "name": "HIP 91582",
+ "pos_x": 35.65625,
+ "pos_y": -55.625,
+ "pos_z": 158.71875,
+ "stations": "Alexander Base,Rusch Camp"
+ },
+ {
+ "name": "HIP 91638",
+ "pos_x": 51.875,
+ "pos_y": -48.125,
+ "pos_z": 97.78125,
+ "stations": "Bahcall Installation,Naboth Plant,Chawla Vision"
+ },
+ {
+ "name": "HIP 91644",
+ "pos_x": 50.46875,
+ "pos_y": -50.53125,
+ "pos_z": 109.8125,
+ "stations": "Houtman Keep,Jones Enterprise"
+ },
+ {
+ "name": "HIP 91645",
+ "pos_x": -74.9375,
+ "pos_y": 8.40625,
+ "pos_z": 102.4375,
+ "stations": "Christopher Colony,Hogan Penal colony"
+ },
+ {
+ "name": "HIP 91701",
+ "pos_x": 73.84375,
+ "pos_y": -67.65625,
+ "pos_z": 134.59375,
+ "stations": "Baynes Installation,Wachmann Park,Dummer Penal colony,Macgregor Landing,Cayley Ring"
+ },
+ {
+ "name": "HIP 91786",
+ "pos_x": 36.34375,
+ "pos_y": -50.9375,
+ "pos_z": 134.09375,
+ "stations": "Camarda Dock,Lundwall Base,Byrd Silo,Pawelczyk Landing"
+ },
+ {
+ "name": "HIP 91805",
+ "pos_x": 25.40625,
+ "pos_y": -49.1875,
+ "pos_z": 146.0625,
+ "stations": "Delporte Hub,Whitney Silo,Wachmann Installation,Anderson Landing"
+ },
+ {
+ "name": "HIP 91818",
+ "pos_x": -113.34375,
+ "pos_y": 16.53125,
+ "pos_z": 133.15625,
+ "stations": "Krylov Horizons"
+ },
+ {
+ "name": "HIP 91837",
+ "pos_x": 64,
+ "pos_y": -74.96875,
+ "pos_z": 178,
+ "stations": "Nouvel Survey,Samuda Terminal"
+ },
+ {
+ "name": "HIP 91857",
+ "pos_x": 27.84375,
+ "pos_y": -54.65625,
+ "pos_z": 161.875,
+ "stations": "Bruce Horizons"
+ },
+ {
+ "name": "HIP 91862",
+ "pos_x": 8.34375,
+ "pos_y": -33.84375,
+ "pos_z": 115.4375,
+ "stations": "Yamazaki Orbital,Coleman City"
+ },
+ {
+ "name": "HIP 91868",
+ "pos_x": -131.53125,
+ "pos_y": 40.21875,
+ "pos_z": 72.125,
+ "stations": "Thagard Survey,Gooch Settlement"
+ },
+ {
+ "name": "HIP 91906",
+ "pos_x": -108.09375,
+ "pos_y": 57.1875,
+ "pos_z": -33.59375,
+ "stations": "Tarski Landing,Crown Port"
+ },
+ {
+ "name": "HIP 9191",
+ "pos_x": 139.6875,
+ "pos_y": -112.09375,
+ "pos_z": 81.34375,
+ "stations": "Sato Terminal,Wisdom Terminal,Paola Holdings"
+ },
+ {
+ "name": "HIP 9216",
+ "pos_x": -0.0625,
+ "pos_y": -170.375,
+ "pos_z": -62.3125,
+ "stations": "Paul Dock,Bode Dock,Goldreich Orbital,Macdonald Survey"
+ },
+ {
+ "name": "HIP 92181",
+ "pos_x": 32.9375,
+ "pos_y": -57.8125,
+ "pos_z": 157.34375,
+ "stations": "Manning Mines"
+ },
+ {
+ "name": "HIP 92342",
+ "pos_x": 49.03125,
+ "pos_y": -49.21875,
+ "pos_z": 98.5,
+ "stations": "Sterling Ring,Rice Orbital,Parkinson Works,Jones Dock,Hynek Depot"
+ },
+ {
+ "name": "HIP 9252",
+ "pos_x": 33.5625,
+ "pos_y": -216.53125,
+ "pos_z": -50.28125,
+ "stations": "Dean Camp,Anderson Works"
+ },
+ {
+ "name": "HIP 92720",
+ "pos_x": -156.03125,
+ "pos_y": 46.96875,
+ "pos_z": 62.71875,
+ "stations": "Besonders Colony,Smith Dock,Houtman Works"
+ },
+ {
+ "name": "HIP 92742",
+ "pos_x": 35.125,
+ "pos_y": -46.75,
+ "pos_z": 105.4375,
+ "stations": "Greene Terminal"
+ },
+ {
+ "name": "HIP 92773",
+ "pos_x": 114.34375,
+ "pos_y": -88.03125,
+ "pos_z": 131.21875,
+ "stations": "Arnold Schwassmann Stop"
+ },
+ {
+ "name": "HIP 92879",
+ "pos_x": -126.53125,
+ "pos_y": 15.71875,
+ "pos_z": 119.84375,
+ "stations": "Altshuller Beacon,Huxley Depot"
+ },
+ {
+ "name": "HIP 92900",
+ "pos_x": -53.0625,
+ "pos_y": -25.34375,
+ "pos_z": 152.96875,
+ "stations": "Marlowe Installation"
+ },
+ {
+ "name": "HIP 92915",
+ "pos_x": 13.65625,
+ "pos_y": -51.5,
+ "pos_z": 147.46875,
+ "stations": "Hyecho Dock,MacVicar Horizons,Wagner Relay"
+ },
+ {
+ "name": "HIP 92916",
+ "pos_x": -132.125,
+ "pos_y": 38.90625,
+ "pos_z": 51.75,
+ "stations": "Ramelli Relay,Leonov Settlement"
+ },
+ {
+ "name": "HIP 92929",
+ "pos_x": -30.5625,
+ "pos_y": -35.5625,
+ "pos_z": 155,
+ "stations": "Read Port,Runco Colony"
+ },
+ {
+ "name": "HIP 92952",
+ "pos_x": -140.9375,
+ "pos_y": 54.34375,
+ "pos_z": 13.3125,
+ "stations": "Anvil Refinery,Dyson Hub,Lewis Point"
+ },
+ {
+ "name": "HIP 93108",
+ "pos_x": -149.75,
+ "pos_y": 50.6875,
+ "pos_z": 33.59375,
+ "stations": "Shonin Orbital,Ivanov Colony"
+ },
+ {
+ "name": "HIP 93119",
+ "pos_x": -113.5625,
+ "pos_y": 57.40625,
+ "pos_z": -33.9375,
+ "stations": "Naddoddur Depot,Buffett Terminal,Baturin City,Howe Port"
+ },
+ {
+ "name": "HIP 9316",
+ "pos_x": 99.65625,
+ "pos_y": -212.28125,
+ "pos_z": 8.5625,
+ "stations": "Millosevich Port,Hulse Gateway,Beriev Dock,Rigaux Station,Trimble Vision,Hogg Port,MacLeod Terminal,Muller Vision,Wisniewski-Snerg Silo,Reed's Progress,Ross Point,Condit Landing,Hutchinson Escape,Barnwell Oasis,Coye Orbital"
+ },
+ {
+ "name": "HIP 93310",
+ "pos_x": -86.375,
+ "pos_y": 4.0625,
+ "pos_z": 93.65625,
+ "stations": "Shosuke's Progress"
+ },
+ {
+ "name": "HIP 93373",
+ "pos_x": 66.9375,
+ "pos_y": -64.96875,
+ "pos_z": 114.4375,
+ "stations": "Kawasato Plant,Plancius City,Bokeili Base"
+ },
+ {
+ "name": "HIP 93377",
+ "pos_x": -93.53125,
+ "pos_y": 9.9375,
+ "pos_z": 83.25,
+ "stations": "Kooi Gateway,Cassidy Horizons,Cayley Horizons,Ostrander's Progress"
+ },
+ {
+ "name": "HIP 9339",
+ "pos_x": -17.09375,
+ "pos_y": -137.46875,
+ "pos_z": -66.5,
+ "stations": "Al-Kashi Legacy"
+ },
+ {
+ "name": "HIP 93427",
+ "pos_x": -124,
+ "pos_y": 36.25,
+ "pos_z": 40.09375,
+ "stations": "Hertz Enterprise,Midgley Mines"
+ },
+ {
+ "name": "HIP 93432",
+ "pos_x": 107.625,
+ "pos_y": -87.5,
+ "pos_z": 132.53125,
+ "stations": "Luther Port,Denning Holdings,Resnick's Folly"
+ },
+ {
+ "name": "HIP 9349",
+ "pos_x": -2.96875,
+ "pos_y": -129.59375,
+ "pos_z": -51.25,
+ "stations": "Lasswitz Mines"
+ },
+ {
+ "name": "HIP 93507",
+ "pos_x": 74.625,
+ "pos_y": -75.5,
+ "pos_z": 135.125,
+ "stations": "Lane Vision,Wallis Hub,Dozois' Inheritance,Gurevich Installation"
+ },
+ {
+ "name": "HIP 93547",
+ "pos_x": 47.4375,
+ "pos_y": -62.59375,
+ "pos_z": 128.3125,
+ "stations": "Noguchi Terminal,Lobachevsky Camp,Pettit Platform"
+ },
+ {
+ "name": "HIP 93566",
+ "pos_x": -106.8125,
+ "pos_y": 11.4375,
+ "pos_z": 90.21875,
+ "stations": "Oltion Relay,Brash Colony,Citroen's Claim"
+ },
+ {
+ "name": "HIP 93639",
+ "pos_x": -20.9375,
+ "pos_y": -37.6875,
+ "pos_z": 133.21875,
+ "stations": "Tereshkova Legacy"
+ },
+ {
+ "name": "HIP 93645",
+ "pos_x": -116.53125,
+ "pos_y": 12.5625,
+ "pos_z": 96.625,
+ "stations": "Shalatula Depot,Carter Landing,Piccard Refinery"
+ },
+ {
+ "name": "HIP 93661",
+ "pos_x": -47.125,
+ "pos_y": -41.21875,
+ "pos_z": 172.875,
+ "stations": "Leichhardt Landing"
+ },
+ {
+ "name": "HIP 93685",
+ "pos_x": -16.75,
+ "pos_y": -49.34375,
+ "pos_z": 161.03125,
+ "stations": "Scott Station,RenenBellot Arsenal"
+ },
+ {
+ "name": "HIP 93690",
+ "pos_x": -87.53125,
+ "pos_y": -22.46875,
+ "pos_z": 163.5,
+ "stations": "Harris Camp"
+ },
+ {
+ "name": "HIP 93745",
+ "pos_x": 73.75,
+ "pos_y": -83.40625,
+ "pos_z": 155.96875,
+ "stations": "Chernykh Platform,Struzan Mines,Carrasco Vista"
+ },
+ {
+ "name": "HIP 93817",
+ "pos_x": -150.0625,
+ "pos_y": 27.1875,
+ "pos_z": 87.71875,
+ "stations": "Burnham Installation,Auld Base"
+ },
+ {
+ "name": "HIP 93827",
+ "pos_x": -50.46875,
+ "pos_y": -19.96875,
+ "pos_z": 111.59375,
+ "stations": "King Station,Whymper Gateway,Kronecker Gateway"
+ },
+ {
+ "name": "HIP 93889",
+ "pos_x": 137.40625,
+ "pos_y": -99.90625,
+ "pos_z": 131.09375,
+ "stations": "Kibalchich Orbital,Williamson Silo,Plancius Station"
+ },
+ {
+ "name": "HIP 9408",
+ "pos_x": 100.78125,
+ "pos_y": -251.375,
+ "pos_z": -6.46875,
+ "stations": "Sekowski Works,Check Orbital,Ejigu Colony"
+ },
+ {
+ "name": "HIP 94126",
+ "pos_x": -164.21875,
+ "pos_y": 81.59375,
+ "pos_z": -52.5625,
+ "stations": "Wilkes Retreat"
+ },
+ {
+ "name": "HIP 9414",
+ "pos_x": 107.34375,
+ "pos_y": -95.34375,
+ "pos_z": 58.6875,
+ "stations": "Mori Station,Karachkina Prospect"
+ },
+ {
+ "name": "HIP 94181",
+ "pos_x": 63.25,
+ "pos_y": -83.8125,
+ "pos_z": 161.5,
+ "stations": "Quetelet Platform,Stiles Outpost"
+ },
+ {
+ "name": "HIP 94184",
+ "pos_x": -22.40625,
+ "pos_y": -52.625,
+ "pos_z": 164.5625,
+ "stations": "Fairbairn Orbital,Silves Installation,White Orbital"
+ },
+ {
+ "name": "HIP 94235",
+ "pos_x": 71.90625,
+ "pos_y": -88.40625,
+ "pos_z": 164.46875,
+ "stations": "Morgan Platform,Covington Survey,Kraft Horizons,Quinn Laboratory"
+ },
+ {
+ "name": "HIP 94252",
+ "pos_x": -158.25,
+ "pos_y": 38.15625,
+ "pos_z": 56.71875,
+ "stations": "Darboux Asylum"
+ },
+ {
+ "name": "HIP 943",
+ "pos_x": -91.09375,
+ "pos_y": -119.3125,
+ "pos_z": -30.03125,
+ "stations": "Lloyd Vision,Erikson Port,Moffitt Lab,Hoyle Bastion"
+ },
+ {
+ "name": "HIP 94326",
+ "pos_x": 3.78125,
+ "pos_y": -53.59375,
+ "pos_z": 138.25,
+ "stations": "Elder Hub,Ibold Arsenal"
+ },
+ {
+ "name": "HIP 94396",
+ "pos_x": -132.8125,
+ "pos_y": 10.3125,
+ "pos_z": 102.28125,
+ "stations": "Laliberte Horizons,Ejeta Terminal,Luiken Observatory"
+ },
+ {
+ "name": "HIP 94417",
+ "pos_x": 36,
+ "pos_y": -76.21875,
+ "pos_z": 164.6875,
+ "stations": "Russell Orbital"
+ },
+ {
+ "name": "HIP 94497",
+ "pos_x": -164.0625,
+ "pos_y": 39.125,
+ "pos_z": 54.84375,
+ "stations": "Fozard Dock,Popper Landing"
+ },
+ {
+ "name": "HIP 94501",
+ "pos_x": -136.28125,
+ "pos_y": 50.53125,
+ "pos_z": -1.21875,
+ "stations": "Savery Ring,Leopold Orbital,Artsebarsky Dock"
+ },
+ {
+ "name": "HIP 9452",
+ "pos_x": -95.4375,
+ "pos_y": -118.46875,
+ "pos_z": -130.21875,
+ "stations": "Ore Terminal,Niven Relay,Mitra Landing"
+ },
+ {
+ "name": "HIP 94570",
+ "pos_x": 55.46875,
+ "pos_y": -76.40625,
+ "pos_z": 144.21875,
+ "stations": "Brera Plant,Tavernier Colony,Haber Enterprise"
+ },
+ {
+ "name": "HIP 94582",
+ "pos_x": -160.84375,
+ "pos_y": 73,
+ "pos_z": -36.75,
+ "stations": "England Platform"
+ },
+ {
+ "name": "HIP 94583",
+ "pos_x": -22.84375,
+ "pos_y": -56.1875,
+ "pos_z": 165.53125,
+ "stations": "Almagro Relay,Chalker Station,Ozanne Dock"
+ },
+ {
+ "name": "HIP 9473",
+ "pos_x": -44.1875,
+ "pos_y": -115.09375,
+ "pos_z": -83.71875,
+ "stations": "Celebi Terminal,Quaglia Orbital,Cousteau Hub,Polansky Works,Galindo's Inheritance"
+ },
+ {
+ "name": "HIP 94832",
+ "pos_x": 21.1875,
+ "pos_y": -75.65625,
+ "pos_z": 169.46875,
+ "stations": "Brillant Penal colony"
+ },
+ {
+ "name": "HIP 94863",
+ "pos_x": 0.03125,
+ "pos_y": -51.1875,
+ "pos_z": 126.8125,
+ "stations": "Grimwood Ring,Napier Station"
+ },
+ {
+ "name": "HIP 94905",
+ "pos_x": -94.96875,
+ "pos_y": 2.46875,
+ "pos_z": 76.84375,
+ "stations": "Gardner Port,Oswald Port,Khan City,Elder Depot"
+ },
+ {
+ "name": "HIP 9492",
+ "pos_x": -78.71875,
+ "pos_y": -103.71875,
+ "pos_z": -110.25,
+ "stations": "Griffith Vision,Ingstad Bastion,Galouye Dock"
+ },
+ {
+ "name": "HIP 94926",
+ "pos_x": -15.28125,
+ "pos_y": -62.78125,
+ "pos_z": 167.6875,
+ "stations": "Rothman Station,Cooper Observatory,Lupoff Terminal"
+ },
+ {
+ "name": "HIP 94939",
+ "pos_x": 15.375,
+ "pos_y": -51.84375,
+ "pos_z": 113.875,
+ "stations": "Barnwell Orbital,Bartolomeu de Gusmao Orbital"
+ },
+ {
+ "name": "HIP 94966",
+ "pos_x": -4.8125,
+ "pos_y": -59.78125,
+ "pos_z": 150.34375,
+ "stations": "Czerny Terminal,Gantt Enterprise,Noriega Base"
+ },
+ {
+ "name": "HIP 9499",
+ "pos_x": 121.875,
+ "pos_y": -170.03125,
+ "pos_z": 42.34375,
+ "stations": "Hogg Orbital,Nylund Installation,Deslandres Port,Sugano Port,Harrison Bastion"
+ },
+ {
+ "name": "HIP 95006",
+ "pos_x": -17.21875,
+ "pos_y": -43.75,
+ "pos_z": 121.25,
+ "stations": "Mayer Station"
+ },
+ {
+ "name": "HIP 95015",
+ "pos_x": -74.28125,
+ "pos_y": -30.90625,
+ "pos_z": 138.625,
+ "stations": "Solovyov Settlement,Howard Works,Mitchell Vision,Vasilyev Holdings"
+ },
+ {
+ "name": "HIP 95044",
+ "pos_x": -151.625,
+ "pos_y": 65.59375,
+ "pos_z": -30.34375,
+ "stations": "Martins Landing,Pook Port"
+ },
+ {
+ "name": "HIP 95055",
+ "pos_x": -89.125,
+ "pos_y": -20.46875,
+ "pos_z": 125.09375,
+ "stations": "Adamson Hub,Teller Dock,Stairs Retreat"
+ },
+ {
+ "name": "HIP 95110",
+ "pos_x": -14.8125,
+ "pos_y": -57.6875,
+ "pos_z": 151.46875,
+ "stations": "Tiptree Colony,al-Haytham Survey,Coelho Survey"
+ },
+ {
+ "name": "HIP 95164",
+ "pos_x": -22.125,
+ "pos_y": -50.15625,
+ "pos_z": 138.3125,
+ "stations": "Rand Keep,Meyrink Orbital,Wul Orbital,Dampier Oasis"
+ },
+ {
+ "name": "HIP 9519",
+ "pos_x": -38.4375,
+ "pos_y": -136.21875,
+ "pos_z": -87.375,
+ "stations": "Al-Jazari Settlement,Locke Platform"
+ },
+ {
+ "name": "HIP 95203",
+ "pos_x": -25,
+ "pos_y": -56.1875,
+ "pos_z": 154.4375,
+ "stations": "Gunn Horizons,Slade Plant,Powers' Inheritance"
+ },
+ {
+ "name": "HIP 95256",
+ "pos_x": 65.75,
+ "pos_y": -70.78125,
+ "pos_z": 113.9375,
+ "stations": "Hansen Station,Baade Vision,Hussenot Terminal,Dall Landing,Bischoff Silo"
+ },
+ {
+ "name": "HIP 95274",
+ "pos_x": -151.0625,
+ "pos_y": 40,
+ "pos_z": 27.8125,
+ "stations": "Ashby Orbital,Roentgen Dock"
+ },
+ {
+ "name": "HIP 95309",
+ "pos_x": -123.25,
+ "pos_y": 5.59375,
+ "pos_z": 85.875,
+ "stations": "J. G. Ballard Terminal,Gaspar de Lemos Vision,Oswald Barracks"
+ },
+ {
+ "name": "HIP 95321",
+ "pos_x": 91.03125,
+ "pos_y": -108.4375,
+ "pos_z": 181.46875,
+ "stations": "Tarter Dock,Verrier Vision,Wallis Terminal"
+ },
+ {
+ "name": "HIP 95350",
+ "pos_x": 54.25,
+ "pos_y": -65.46875,
+ "pos_z": 109.90625,
+ "stations": "Oikawa Station,Ludwig Struve Orbital,Niemeyer Orbital,Baird's Progress,Ferguson Orbital,Fraknoi Port,Buckell's Folly,Perrine Vision"
+ },
+ {
+ "name": "HIP 95457",
+ "pos_x": -41.4375,
+ "pos_y": -49.28125,
+ "pos_z": 146.25,
+ "stations": "Ocampo Refinery,Cook Survey,Lebesgue Installation"
+ },
+ {
+ "name": "HIP 955",
+ "pos_x": -43.4375,
+ "pos_y": -190.15625,
+ "pos_z": 7.65625,
+ "stations": "Turner Depot,Moore Prospect"
+ },
+ {
+ "name": "HIP 95532",
+ "pos_x": 36.25,
+ "pos_y": -88.1875,
+ "pos_z": 174.375,
+ "stations": "Charlier Terminal,Searle Port,Baynes Port,Jordan Relay,RenenBellot Enterprise"
+ },
+ {
+ "name": "HIP 95640",
+ "pos_x": 116.28125,
+ "pos_y": -107.09375,
+ "pos_z": 155.25,
+ "stations": "Wang Vision,van Houten Colony,Poncelet Hub"
+ },
+ {
+ "name": "HIP 95641",
+ "pos_x": -11.3125,
+ "pos_y": -59.3125,
+ "pos_z": 142.78125,
+ "stations": "Otto Landing"
+ },
+ {
+ "name": "HIP 95677",
+ "pos_x": -61.53125,
+ "pos_y": -52.34375,
+ "pos_z": 163.875,
+ "stations": "Maclaurin Installation"
+ },
+ {
+ "name": "HIP 95720",
+ "pos_x": 140.03125,
+ "pos_y": -126.28125,
+ "pos_z": 180.03125,
+ "stations": "Arend Installation"
+ },
+ {
+ "name": "HIP 95772",
+ "pos_x": -108.65625,
+ "pos_y": -9.25,
+ "pos_z": 99.96875,
+ "stations": "Shunn Station"
+ },
+ {
+ "name": "HIP 95864",
+ "pos_x": -5.0625,
+ "pos_y": -59.90625,
+ "pos_z": 135.96875,
+ "stations": "Klimuk Dock,Midgley Colony,Cabana Hub,Amis Lab"
+ },
+ {
+ "name": "HIP 95938",
+ "pos_x": -164.6875,
+ "pos_y": 23.75,
+ "pos_z": 64.125,
+ "stations": "Jernigan Terminal,Descartes Enterprise,Webb's Folly"
+ },
+ {
+ "name": "HIP 95973",
+ "pos_x": -87,
+ "pos_y": -48.84375,
+ "pos_z": 167.875,
+ "stations": "Burnham Dock,Scalzi Station"
+ },
+ {
+ "name": "HIP 9599",
+ "pos_x": -41.6875,
+ "pos_y": -98.59375,
+ "pos_z": -76.375,
+ "stations": "Guidoni Enterprise,Rescue Ship - Guidoni Enterprise"
+ },
+ {
+ "name": "HIP 96037",
+ "pos_x": -131.5625,
+ "pos_y": -2.59375,
+ "pos_z": 96.875,
+ "stations": "Ron Hubbard Relay"
+ },
+ {
+ "name": "HIP 9607",
+ "pos_x": -56.125,
+ "pos_y": -115.8125,
+ "pos_z": -96.21875,
+ "stations": "Lukyanenko Dock"
+ },
+ {
+ "name": "HIP 96072",
+ "pos_x": -102.1875,
+ "pos_y": -18.4375,
+ "pos_z": 110.3125,
+ "stations": "Hoyle Hub,Bester City,Barentsz's Inheritance"
+ },
+ {
+ "name": "HIP 96077",
+ "pos_x": -158.65625,
+ "pos_y": 40.8125,
+ "pos_z": 20.84375,
+ "stations": "Soto Camp,Farouk Mines,Wafer Keep"
+ },
+ {
+ "name": "HIP 96095",
+ "pos_x": 12.125,
+ "pos_y": -59.9375,
+ "pos_z": 121.15625,
+ "stations": "Tepper Enterprise,Kennicott Installation,Bacigalupi Plant,Mouhot Landing,Moorcock Platform"
+ },
+ {
+ "name": "HIP 9610",
+ "pos_x": 176.9375,
+ "pos_y": -160.625,
+ "pos_z": 94.5625,
+ "stations": "Carpenter Colony,Tolstoi Holdings"
+ },
+ {
+ "name": "HIP 96160",
+ "pos_x": 44.1875,
+ "pos_y": -82.59375,
+ "pos_z": 147.125,
+ "stations": "Fiennes Oasis,Yu Vision,Bisson Vision"
+ },
+ {
+ "name": "HIP 96184",
+ "pos_x": -122.40625,
+ "pos_y": 3.78125,
+ "pos_z": 74.0625,
+ "stations": "Fisher Colony,Schottky Terminal,Steinmuller Depot"
+ },
+ {
+ "name": "HIP 96240",
+ "pos_x": -42.6875,
+ "pos_y": -53.75,
+ "pos_z": 142.40625,
+ "stations": "Leibniz Port,Weiss Bastion"
+ },
+ {
+ "name": "HIP 96273",
+ "pos_x": -40.34375,
+ "pos_y": -72.71875,
+ "pos_z": 180.375,
+ "stations": "Greenleaf Orbital"
+ },
+ {
+ "name": "HIP 96289",
+ "pos_x": 124.34375,
+ "pos_y": -115.21875,
+ "pos_z": 161.75,
+ "stations": "Grigorovich Camp,Pryor Penal colony"
+ },
+ {
+ "name": "HIP 96308",
+ "pos_x": -101.78125,
+ "pos_y": -11.21875,
+ "pos_z": 90.15625,
+ "stations": "Baird Ring,Phillips Orbital,Linteris Orbital,Stevens Hub,Quimby Terminal"
+ },
+ {
+ "name": "HIP 96345",
+ "pos_x": 73.90625,
+ "pos_y": -76.40625,
+ "pos_z": 112.53125,
+ "stations": "Metcalf Dock,Sugano Terminal,Arisman Orbital"
+ },
+ {
+ "name": "HIP 96402",
+ "pos_x": -77.1875,
+ "pos_y": -22.6875,
+ "pos_z": 96.8125,
+ "stations": "Dutton Terminal,Drew Station,Ehrlich Market,Macdonald Vision,Ivanov Hub,Crippen Hub"
+ },
+ {
+ "name": "HIP 96456",
+ "pos_x": -208.4375,
+ "pos_y": -1.78125,
+ "pos_z": 135.84375,
+ "stations": "Lemmy's Rock"
+ },
+ {
+ "name": "HIP 96487",
+ "pos_x": 110.78125,
+ "pos_y": -112.65625,
+ "pos_z": 163.40625,
+ "stations": "Lopez-Garcia Settlement"
+ },
+ {
+ "name": "HIP 9649",
+ "pos_x": 108.09375,
+ "pos_y": -171.75,
+ "pos_z": 28.21875,
+ "stations": "Koishikawa Hub,Cheranovsky Horizons"
+ },
+ {
+ "name": "HIP 96515",
+ "pos_x": 28.90625,
+ "pos_y": -68.0625,
+ "pos_z": 122.5,
+ "stations": "Kozin Point"
+ },
+ {
+ "name": "HIP 9659",
+ "pos_x": -104.5,
+ "pos_y": -2.5,
+ "pos_z": -94.78125,
+ "stations": "Shinn City,Lessing Survey,Aitken Relay"
+ },
+ {
+ "name": "HIP 9672",
+ "pos_x": 85.25,
+ "pos_y": -210.375,
+ "pos_z": -8.125,
+ "stations": "Parker Orbital,Kushida Dock,Faber Orbital,Barr Observatory,Heck Installation,Brule Retreat"
+ },
+ {
+ "name": "HIP 96725",
+ "pos_x": 21.375,
+ "pos_y": -70.15625,
+ "pos_z": 128.84375,
+ "stations": "Brahmagupta Vision"
+ },
+ {
+ "name": "HIP 96834",
+ "pos_x": -93.09375,
+ "pos_y": -33.65625,
+ "pos_z": 121.90625,
+ "stations": "Coblentz Horizons,Resnick Hub"
+ },
+ {
+ "name": "HIP 96854",
+ "pos_x": -16.3125,
+ "pos_y": -59.4375,
+ "pos_z": 128,
+ "stations": "Dowie Port,Mitra Barracks"
+ },
+ {
+ "name": "HIP 96880",
+ "pos_x": 73.21875,
+ "pos_y": -69.9375,
+ "pos_z": 96.5625,
+ "stations": "Lyulka Ring,Curtiss Port,Flammarion Orbital,Naubakht Refinery"
+ },
+ {
+ "name": "HIP 96906",
+ "pos_x": -160.03125,
+ "pos_y": 18.375,
+ "pos_z": 55.625,
+ "stations": "Camarda Port"
+ },
+ {
+ "name": "HIP 96932",
+ "pos_x": -7.53125,
+ "pos_y": -63.0625,
+ "pos_z": 128.9375,
+ "stations": "Bond Dock,Simak Penal colony,Al-Kashi's Inheritance"
+ },
+ {
+ "name": "HIP 96943",
+ "pos_x": -113.0625,
+ "pos_y": -21.71875,
+ "pos_z": 107.25,
+ "stations": "Shirley City,Pytheas Installation,Vries Installation"
+ },
+ {
+ "name": "HIP 97024",
+ "pos_x": 134.96875,
+ "pos_y": -126.46875,
+ "pos_z": 172.0625,
+ "stations": "Ilyushin Enterprise,Crossfield Dock,Riazuddin Prospect"
+ },
+ {
+ "name": "HIP 97048",
+ "pos_x": -117.0625,
+ "pos_y": -1.21875,
+ "pos_z": 67.9375,
+ "stations": "Bosch Settlement,Melvill Camp,Williams Works"
+ },
+ {
+ "name": "HIP 97096",
+ "pos_x": 88.96875,
+ "pos_y": -104.25,
+ "pos_z": 153.65625,
+ "stations": "Goryu Hub,Addams' Claim"
+ },
+ {
+ "name": "HIP 97160",
+ "pos_x": 0.90625,
+ "pos_y": -66.90625,
+ "pos_z": 128.71875,
+ "stations": "Smith's Pride,Herbert Base"
+ },
+ {
+ "name": "HIP 97174",
+ "pos_x": -158.09375,
+ "pos_y": 6.59375,
+ "pos_z": 73.15625,
+ "stations": "Haarsma Gateway,Willis Port,Strzelecki Arsenal,Bunch Stop"
+ },
+ {
+ "name": "HIP 97185",
+ "pos_x": 111.5625,
+ "pos_y": -115.9375,
+ "pos_z": 163,
+ "stations": "Kraft Mines,Carroll Works"
+ },
+ {
+ "name": "HIP 97196",
+ "pos_x": 70.78125,
+ "pos_y": -77.5625,
+ "pos_z": 111.0625,
+ "stations": "Gurshtein Dock,Conti Relay,van den Bergh Settlement"
+ },
+ {
+ "name": "HIP 97336",
+ "pos_x": -147.28125,
+ "pos_y": 12.09375,
+ "pos_z": 54.25,
+ "stations": "Silves Terminal"
+ },
+ {
+ "name": "HIP 97337",
+ "pos_x": -115.375,
+ "pos_y": 15.40625,
+ "pos_z": 31.03125,
+ "stations": "Denton Dock,Bolger Installation"
+ },
+ {
+ "name": "HIP 97384",
+ "pos_x": -100.53125,
+ "pos_y": -33.9375,
+ "pos_z": 116.28125,
+ "stations": "Eilenberg Gateway,Dowling Orbital,Hiroyuki Prospect"
+ },
+ {
+ "name": "HIP 97399",
+ "pos_x": -169.96875,
+ "pos_y": 56.53125,
+ "pos_z": -18.875,
+ "stations": "Pelliot Dock,Lorrah Orbital"
+ },
+ {
+ "name": "HIP 9741",
+ "pos_x": 104.78125,
+ "pos_y": -190.28125,
+ "pos_z": 16.8125,
+ "stations": "d'Arrest Terminal,Chertok Port,Tedin Hub,De Lay Relay,Helffrich Terminal"
+ },
+ {
+ "name": "HIP 9742",
+ "pos_x": -29.9375,
+ "pos_y": -131.0625,
+ "pos_z": -80.5,
+ "stations": "Helms Park,Messerschmid Landing,Aubakirov Port,Park Vision"
+ },
+ {
+ "name": "HIP 97507",
+ "pos_x": 85.8125,
+ "pos_y": -89.8125,
+ "pos_z": 124.3125,
+ "stations": "Hickam Hub,Roelofs Orbital,Matthaus Olbers Colony,Kingsbury Beacon"
+ },
+ {
+ "name": "HIP 97508",
+ "pos_x": 68.3125,
+ "pos_y": -97.5,
+ "pos_z": 147.4375,
+ "stations": "Sopwith Dock,Brouwer Relay"
+ },
+ {
+ "name": "HIP 97509",
+ "pos_x": -148,
+ "pos_y": 1.6875,
+ "pos_z": 71.34375,
+ "stations": "Hamilton Colony,Moon Base"
+ },
+ {
+ "name": "HIP 9753",
+ "pos_x": -7.40625,
+ "pos_y": -116.90625,
+ "pos_z": -54.46875,
+ "stations": "Harawi Enterprise,Chiang Ring,Armero Settlement"
+ },
+ {
+ "name": "HIP 97531",
+ "pos_x": -17.4375,
+ "pos_y": -63.5,
+ "pos_z": 126.9375,
+ "stations": "Ray Terminal,Hieb Plant"
+ },
+ {
+ "name": "HIP 97537",
+ "pos_x": -133.375,
+ "pos_y": 2.5,
+ "pos_z": 62.0625,
+ "stations": "Shapiro Orbital,Hedin Terminal,Hartog Orbital,Archer Barracks"
+ },
+ {
+ "name": "HIP 97546",
+ "pos_x": -5.625,
+ "pos_y": -66.84375,
+ "pos_z": 127,
+ "stations": "Bohr Vision,Miklouho-Maclay Plant"
+ },
+ {
+ "name": "HIP 97548",
+ "pos_x": 50.375,
+ "pos_y": -99.9375,
+ "pos_z": 160.5,
+ "stations": "Becvar Base,Ackerman's Inheritance"
+ },
+ {
+ "name": "HIP 97564",
+ "pos_x": 85.875,
+ "pos_y": -108.4375,
+ "pos_z": 158.375,
+ "stations": "Weierstrass Arena,Palitzsch Station,Foglio Port"
+ },
+ {
+ "name": "HIP 97567",
+ "pos_x": 8.4375,
+ "pos_y": -75.96875,
+ "pos_z": 136.65625,
+ "stations": "Baker Port,Galilei Port,Stein Arena"
+ },
+ {
+ "name": "HIP 9764",
+ "pos_x": -57.3125,
+ "pos_y": -122.25,
+ "pos_z": -101.8125,
+ "stations": "Pausch Camp,Coulomb Port"
+ },
+ {
+ "name": "HIP 9768",
+ "pos_x": 134.84375,
+ "pos_y": -251.09375,
+ "pos_z": 18.78125,
+ "stations": "Brooks Settlement,Filippenko Survey,Hodgkinson Terminal"
+ },
+ {
+ "name": "HIP 9769",
+ "pos_x": 19.71875,
+ "pos_y": -136.40625,
+ "pos_z": -38.15625,
+ "stations": "Nicolet Arsenal,Asclepi Terminal"
+ },
+ {
+ "name": "HIP 97704",
+ "pos_x": -90.34375,
+ "pos_y": -37.28125,
+ "pos_z": 111.84375,
+ "stations": "al-Haytham Vista,Harrison Works"
+ },
+ {
+ "name": "HIP 97705",
+ "pos_x": 67.84375,
+ "pos_y": -114.4375,
+ "pos_z": 176.8125,
+ "stations": "Westerhout's Exile,Duque Installation"
+ },
+ {
+ "name": "HIP 9774",
+ "pos_x": 21,
+ "pos_y": -145.09375,
+ "pos_z": -40.625,
+ "stations": "Zoline Lab,Thomas Terminal,McDevitt Settlement"
+ },
+ {
+ "name": "HIP 97940",
+ "pos_x": -96.5625,
+ "pos_y": -34.40625,
+ "pos_z": 105.625,
+ "stations": "Eudoxus Observatory"
+ },
+ {
+ "name": "HIP 97950",
+ "pos_x": -91.34375,
+ "pos_y": -32.625,
+ "pos_z": 99.84375,
+ "stations": "Hoffman Installation,Sellings Holdings,Hausdorff Holdings"
+ },
+ {
+ "name": "HIP 97977",
+ "pos_x": -20.40625,
+ "pos_y": -79.0625,
+ "pos_z": 150.15625,
+ "stations": "Palmer City,Verne Ring,Sarrantonio Orbital,Coney Depot"
+ },
+ {
+ "name": "HIP 98001",
+ "pos_x": -145.90625,
+ "pos_y": 18,
+ "pos_z": 33.625,
+ "stations": "Shull Terminal,Macarthur Hub"
+ },
+ {
+ "name": "HIP 98020",
+ "pos_x": -92.78125,
+ "pos_y": -19.875,
+ "pos_z": 76.875,
+ "stations": "Pogue Colony,Anderson Colony"
+ },
+ {
+ "name": "HIP 98049",
+ "pos_x": -32.1875,
+ "pos_y": -75.3125,
+ "pos_z": 147.71875,
+ "stations": "Doyle's Folly"
+ },
+ {
+ "name": "HIP 98211",
+ "pos_x": 34.8125,
+ "pos_y": -71,
+ "pos_z": 108.875,
+ "stations": "Lukyanenko Dock,Baker Landing,Hume Survey"
+ },
+ {
+ "name": "HIP 98237",
+ "pos_x": -57.15625,
+ "pos_y": -74.5,
+ "pos_z": 153.90625,
+ "stations": "Narvaez Base"
+ },
+ {
+ "name": "HIP 98274",
+ "pos_x": 22.96875,
+ "pos_y": -91,
+ "pos_z": 148.21875,
+ "stations": "Bingzhen Port,Borrelly Landing,Kirshner Platform,Thompson Works,Hume Refinery"
+ },
+ {
+ "name": "HIP 98381",
+ "pos_x": -131.78125,
+ "pos_y": 14.9375,
+ "pos_z": 28.71875,
+ "stations": "Herschel Gateway,Bridger Settlement"
+ },
+ {
+ "name": "HIP 98451",
+ "pos_x": 20.53125,
+ "pos_y": -97.28125,
+ "pos_z": 157.75,
+ "stations": "Marsden Mine"
+ },
+ {
+ "name": "HIP 9846",
+ "pos_x": 119.5625,
+ "pos_y": -261.875,
+ "pos_z": -0.75,
+ "stations": "Lyot Stop"
+ },
+ {
+ "name": "HIP 98621",
+ "pos_x": 57.6875,
+ "pos_y": -65.03125,
+ "pos_z": 86.9375,
+ "stations": "Larson Lab,Kapteyn Port,Fox Dock,Lukyanenko Lab,Tucker Platform,Banno Station,Lintott Vision,Ziemianski Prospect,MacCurdy Bastion,Herbig Terminal,Ejigu Terminal,Rucker's Progress,Ordway Port"
+ },
+ {
+ "name": "HIP 98704",
+ "pos_x": 132.34375,
+ "pos_y": -122.3125,
+ "pos_z": 153.6875,
+ "stations": "Cowling's Inheritance,Schoening Prospect"
+ },
+ {
+ "name": "HIP 98723",
+ "pos_x": -72.8125,
+ "pos_y": -43.5,
+ "pos_z": 100.46875,
+ "stations": "Herreshoff Survey,Butcher Dock"
+ },
+ {
+ "name": "HIP 98839",
+ "pos_x": -37.09375,
+ "pos_y": -70.1875,
+ "pos_z": 129.9375,
+ "stations": "Gaspar de Lemos Station,Zahn Station"
+ },
+ {
+ "name": "HIP 98878",
+ "pos_x": -81.78125,
+ "pos_y": -34.8125,
+ "pos_z": 87.5625,
+ "stations": "Baxter's Exile"
+ },
+ {
+ "name": "HIP 98879",
+ "pos_x": -110.21875,
+ "pos_y": -20.3125,
+ "pos_z": 74.1875,
+ "stations": "Kimbrough Vision,Guest Dock"
+ },
+ {
+ "name": "HIP 98978",
+ "pos_x": -161.28125,
+ "pos_y": 24.96875,
+ "pos_z": 17.59375,
+ "stations": "Pordenone Plant,Phillpotts Horizons"
+ },
+ {
+ "name": "HIP 99034",
+ "pos_x": -73.4375,
+ "pos_y": -69,
+ "pos_z": 138.53125,
+ "stations": "Bowen Station,Herrington Settlement,Robinson Vision"
+ },
+ {
+ "name": "HIP 99046",
+ "pos_x": -48.625,
+ "pos_y": -67.125,
+ "pos_z": 126.46875,
+ "stations": "Elder Relay,Stross Depot,Holub Beacon"
+ },
+ {
+ "name": "HIP 99139",
+ "pos_x": 0.65625,
+ "pos_y": -95.84375,
+ "pos_z": 154.375,
+ "stations": "Karman Settlement,Narlikar Keep"
+ },
+ {
+ "name": "HIP 99188",
+ "pos_x": 36.625,
+ "pos_y": -100.1875,
+ "pos_z": 148.34375,
+ "stations": "Nikitin Survey,Hildebrandt Port,Sy Platform"
+ },
+ {
+ "name": "HIP 9921",
+ "pos_x": 128.28125,
+ "pos_y": -235.4375,
+ "pos_z": 17.125,
+ "stations": "Curtiss Penal colony,Moore Survey"
+ },
+ {
+ "name": "HIP 99210",
+ "pos_x": -148.875,
+ "pos_y": -30.15625,
+ "pos_z": 99.1875,
+ "stations": "Wiener's Folly"
+ },
+ {
+ "name": "HIP 99224",
+ "pos_x": -80.25,
+ "pos_y": -79.875,
+ "pos_z": 155.3125,
+ "stations": "Meyrink Enterprise"
+ },
+ {
+ "name": "HIP 99241",
+ "pos_x": -145.28125,
+ "pos_y": 24.125,
+ "pos_z": 10.71875,
+ "stations": "Beadle Ring,Kovalyonok City,Waldrop Installation"
+ },
+ {
+ "name": "HIP 99271",
+ "pos_x": 130.40625,
+ "pos_y": -126.59375,
+ "pos_z": 158.0625,
+ "stations": "Freas Enterprise,Bradley Depot,Scheerbart Depot"
+ },
+ {
+ "name": "HIP 99344",
+ "pos_x": 23.6875,
+ "pos_y": -70.5625,
+ "pos_z": 104.125,
+ "stations": "Banno Escape"
+ },
+ {
+ "name": "HIP 99351",
+ "pos_x": -126.34375,
+ "pos_y": -17.84375,
+ "pos_z": 70.0625,
+ "stations": "Rond d'Alembert Landing"
+ },
+ {
+ "name": "HIP 99397",
+ "pos_x": 32.53125,
+ "pos_y": -83.25,
+ "pos_z": 120.84375,
+ "stations": "Oikawa City,Sagan Point,Pimi City,Harvey-Smith Vision"
+ },
+ {
+ "name": "HIP 99551",
+ "pos_x": 155.84375,
+ "pos_y": -139.53125,
+ "pos_z": 168.5625,
+ "stations": "Hynek's Inheritance"
+ },
+ {
+ "name": "HIP 99591",
+ "pos_x": -19.84375,
+ "pos_y": -91.0625,
+ "pos_z": 147.65625,
+ "stations": "Milne Terminal,Reinmuth Prospect,Aitken City,Ross' Folly,Ford Base"
+ },
+ {
+ "name": "HIP 996",
+ "pos_x": -122.21875,
+ "pos_y": -116.46875,
+ "pos_z": -48.21875,
+ "stations": "Bryusov Terminal,Beaufoy Hub,Conti Port"
+ },
+ {
+ "name": "HIP 99606",
+ "pos_x": -13.375,
+ "pos_y": -67.03125,
+ "pos_z": 108.15625,
+ "stations": "Levinson Vision,Gentle's Pride,Tago Barracks"
+ },
+ {
+ "name": "HIP 99642",
+ "pos_x": -11,
+ "pos_y": -72.25,
+ "pos_z": 115.09375,
+ "stations": "Unsold Port,Potocnik Port,Stevenson Terminal"
+ },
+ {
+ "name": "HIP 99680",
+ "pos_x": -142.28125,
+ "pos_y": 25.40625,
+ "pos_z": 4.0625,
+ "stations": "Hubble Gateway,Rennie Gateway,Pournelle Survey,Kuttner Landing"
+ },
+ {
+ "name": "HIP 99695",
+ "pos_x": 18.59375,
+ "pos_y": -91.21875,
+ "pos_z": 134.90625,
+ "stations": "Burnham Survey,Allen Prospect,Bohrmann Station,Wilson Enterprise"
+ },
+ {
+ "name": "HIP 9971",
+ "pos_x": -93.34375,
+ "pos_y": -94.625,
+ "pos_z": -125.875,
+ "stations": "Maybury Beacon,Isherwood Installation"
+ },
+ {
+ "name": "HIP 99737",
+ "pos_x": -196.75,
+ "pos_y": 0.65625,
+ "pos_z": 58.0625,
+ "stations": "Clark Hub,Coppel Vision"
+ },
+ {
+ "name": "HIP 99792",
+ "pos_x": -119.75,
+ "pos_y": -22.875,
+ "pos_z": 70.40625,
+ "stations": "Hieb Settlement,Guidoni Stop,Zindell's Progress"
+ },
+ {
+ "name": "HIP 9989",
+ "pos_x": -21.65625,
+ "pos_y": -105,
+ "pos_z": -64.96875,
+ "stations": "Capek Orbital,Caselberg Hub,Regiomontanus Ring,Flammarion's Inheritance"
+ },
+ {
+ "name": "HIP 99902",
+ "pos_x": 138.375,
+ "pos_y": -101,
+ "pos_z": 113.28125,
+ "stations": "Pacheco Mines,Hollander Enterprise,Meech Platform,Penrose Depot,Lupoff Horizons"
+ },
+ {
+ "name": "HIP 99940",
+ "pos_x": -15.21875,
+ "pos_y": -78.25,
+ "pos_z": 122.46875,
+ "stations": "Antonelli Enterprise,Fleming Enterprise,Fettman City,Noguchi Hub,Duffy Orbital,Gloss Works"
+ },
+ {
+ "name": "HIP 99945",
+ "pos_x": 50.78125,
+ "pos_y": -93.90625,
+ "pos_z": 127.3125,
+ "stations": "Nomen Station,Danforth Orbital,Tuttle Port,Johnson Terminal"
+ },
+ {
+ "name": "HIP 99957",
+ "pos_x": -0.5625,
+ "pos_y": -90,
+ "pos_z": 135.8125,
+ "stations": "Ptack Survey,Urata Prospect"
+ },
+ {
+ "name": "HIP 99966",
+ "pos_x": -18.8125,
+ "pos_y": -68.03125,
+ "pos_z": 107.75,
+ "stations": "Blenkinsop Point,Roberts Terminal"
+ },
+ {
+ "name": "HIP 99971",
+ "pos_x": -67.78125,
+ "pos_y": -56.59375,
+ "pos_z": 104.34375,
+ "stations": "Haldeman II Terminal,Haberlandt Holdings"
+ },
+ {
+ "name": "HIP 99994",
+ "pos_x": 106.5,
+ "pos_y": -134.25,
+ "pos_z": 172.0625,
+ "stations": "Schoenherr Depot,Cannon Terminal"
+ },
+ {
+ "name": "Hira",
+ "pos_x": 39.75,
+ "pos_y": -200.8125,
+ "pos_z": 59.875,
+ "stations": "van der Riet Woolley Reformatory"
+ },
+ {
+ "name": "Hiradha",
+ "pos_x": 29.03125,
+ "pos_y": -164.0625,
+ "pos_z": -65.90625,
+ "stations": "Babcock Base,Kraft Survey,Phillips Beacon"
+ },
+ {
+ "name": "Hiradhea",
+ "pos_x": 74.875,
+ "pos_y": -8.5,
+ "pos_z": -9.5625,
+ "stations": "Irwin Survey,Cogswell Settlement,Magnus Hub"
+ },
+ {
+ "name": "Hiraja",
+ "pos_x": -15.375,
+ "pos_y": -189.90625,
+ "pos_z": 61.875,
+ "stations": "Kerimov's Claim,Flaugergues Point"
+ },
+ {
+ "name": "Hiralangi",
+ "pos_x": -67.84375,
+ "pos_y": 12.78125,
+ "pos_z": -76.71875,
+ "stations": "Greenland Arena,Champlain's Progress,Wandrei Installation"
+ },
+ {
+ "name": "Hiralarsh",
+ "pos_x": 43.15625,
+ "pos_y": 104.90625,
+ "pos_z": 23.34375,
+ "stations": "Margulis Gateway,Richards City,Pogue Port,Carrier Beacon"
+ },
+ {
+ "name": "Hirani",
+ "pos_x": -110.25,
+ "pos_y": 51.53125,
+ "pos_z": 40.1875,
+ "stations": "Teller City,Morey City,Leavitt Enterprise,Howe Holdings"
+ },
+ {
+ "name": "Hiranyaksha",
+ "pos_x": -124.625,
+ "pos_y": -60.25,
+ "pos_z": 4.65625,
+ "stations": "Wood Ring,Watts Ring,Akers Horizons,Fast Port"
+ },
+ {
+ "name": "Hirapa",
+ "pos_x": 121.34375,
+ "pos_y": -88.8125,
+ "pos_z": 5.8125,
+ "stations": "Payne-Gaposchkin Port,Kowal Port,Peltier Hub,Banno Ring,Hubble Vision,McDaniel Palace,Woodroffe Terminal,Roth Terminal,Butler Works"
+ },
+ {
+ "name": "Hirarimpt",
+ "pos_x": 18.875,
+ "pos_y": 155.53125,
+ "pos_z": 47.4375,
+ "stations": "Stokes Station,Rasmussen Enterprise,Smith Base,Bruce Bastion"
+ },
+ {
+ "name": "Hirata",
+ "pos_x": -7.375,
+ "pos_y": -133.25,
+ "pos_z": 115.53125,
+ "stations": "Eisinga Beacon"
+ },
+ {
+ "name": "Hirpasuk",
+ "pos_x": 123.90625,
+ "pos_y": -49.3125,
+ "pos_z": 75,
+ "stations": "Langley Station,Endate City"
+ },
+ {
+ "name": "Hirpinyi",
+ "pos_x": -168.40625,
+ "pos_y": -23.5,
+ "pos_z": 41.125,
+ "stations": "Altshuller Gateway,Leiber Hub,Ross Dock,Hawkes Terminal,Grigson Base"
+ },
+ {
+ "name": "Hisaaka",
+ "pos_x": 122.375,
+ "pos_y": -99.5,
+ "pos_z": -15.1875,
+ "stations": "Kahn Vision"
+ },
+ {
+ "name": "Hiwi",
+ "pos_x": 144.1875,
+ "pos_y": 69.84375,
+ "pos_z": 48.875,
+ "stations": "Knapp Platform,Pauli Colony,Fancher Hub"
+ },
+ {
+ "name": "Hixkar",
+ "pos_x": -96.8125,
+ "pos_y": -5.4375,
+ "pos_z": -2.4375,
+ "stations": "Dobrovolski Gateway,Loncke Depot,Snyder Hub,Elwood Lab"
+ },
+ {
+ "name": "Hixkaramu",
+ "pos_x": 115.625,
+ "pos_y": -48.59375,
+ "pos_z": -58.28125,
+ "stations": "Huygens Hub,Heng Orbital,Faris Terminal,Gooch Station,Bartlett Holdings,Leonov Hub,Foden Exchange,Hauck Town,Vogel Port,Laveykin Orbital,Back Survey"
+ },
+ {
+ "name": "Hixkariansi",
+ "pos_x": 69.46875,
+ "pos_y": -116.53125,
+ "pos_z": 128.28125,
+ "stations": "Iwamoto Landing,Gold Hub"
+ },
+ {
+ "name": "Hixkarines",
+ "pos_x": -17.375,
+ "pos_y": -3.75,
+ "pos_z": -140.40625,
+ "stations": "Rzeppa Colony,Doyle Landing"
+ },
+ {
+ "name": "Hixkaryak",
+ "pos_x": 76.09375,
+ "pos_y": -177.78125,
+ "pos_z": -46.8125,
+ "stations": "Oosterhoff Vision,Babcock Hub,Willis Point,Frost's Progress,Duque Terminal,Paczynski Orbital,Curtiss Terminal"
+ },
+ {
+ "name": "HK Aquarii",
+ "pos_x": -26.34375,
+ "pos_y": -65.1875,
+ "pos_z": 18.40625,
+ "stations": "Leestma Enterprise,Torricelli Enterprise,Dunn Laboratory,Usachov Dock"
+ },
+ {
+ "name": "Hladaragua",
+ "pos_x": 73.4375,
+ "pos_y": -181.28125,
+ "pos_z": 0.21875,
+ "stations": "Brin Survey,Jansky Point"
+ },
+ {
+ "name": "Hladgunnos",
+ "pos_x": -107.34375,
+ "pos_y": 8.125,
+ "pos_z": 31.65625,
+ "stations": "Meredith Hub,King Gateway,Wrangel Prospect,Solovyev Relay"
+ },
+ {
+ "name": "Hlin",
+ "pos_x": -41.28125,
+ "pos_y": 6.4375,
+ "pos_z": -58.3125,
+ "stations": "Tull Hub,Dunbar Colony"
+ },
+ {
+ "name": "Hloca",
+ "pos_x": 16.625,
+ "pos_y": -179.53125,
+ "pos_z": 3.375,
+ "stations": "Barlowe Dock,Hornoch Port"
+ },
+ {
+ "name": "Hlocama",
+ "pos_x": 100.9375,
+ "pos_y": 79.4375,
+ "pos_z": -31.1875,
+ "stations": "Tapinas Hub"
+ },
+ {
+ "name": "Hlocamentii",
+ "pos_x": 98.3125,
+ "pos_y": 45.21875,
+ "pos_z": 99.3125,
+ "stations": "Chorel Mine,Kerr Prospect,Pribylov Relay"
+ },
+ {
+ "name": "Hlocasses",
+ "pos_x": 149.8125,
+ "pos_y": -209.21875,
+ "pos_z": 46.1875,
+ "stations": "Weill Hub,Nakamura Platform"
+ },
+ {
+ "name": "Hloch",
+ "pos_x": 5.53125,
+ "pos_y": -103.21875,
+ "pos_z": 93.5,
+ "stations": "Leoniceno Prospect"
+ },
+ {
+ "name": "Hlocidirus",
+ "pos_x": 30.96875,
+ "pos_y": 19.78125,
+ "pos_z": -29.78125,
+ "stations": "Reynolds City"
+ },
+ {
+ "name": "Hlock Ek",
+ "pos_x": 143.6875,
+ "pos_y": -41.34375,
+ "pos_z": -16.75,
+ "stations": "Minkowski Retreat"
+ },
+ {
+ "name": "HM Bootis",
+ "pos_x": -44,
+ "pos_y": 109.65625,
+ "pos_z": 18.8125,
+ "stations": "Fernandez Co-operative,Nesvadba Point,Hutchinson Gateway"
+ },
+ {
+ "name": "Hmar",
+ "pos_x": -41.65625,
+ "pos_y": 58.46875,
+ "pos_z": 71.90625,
+ "stations": "Wiley Terminal,Reynolds Terminal,Bosch Enterprise,Harris Relay,Howe City"
+ },
+ {
+ "name": "Hmargk",
+ "pos_x": 36.40625,
+ "pos_y": -22.5,
+ "pos_z": 76.1875,
+ "stations": "McKay Dock,Swainson Station,Kaku Point"
+ },
+ {
+ "name": "Hmaridge",
+ "pos_x": -52.71875,
+ "pos_y": -147.625,
+ "pos_z": 78.46875,
+ "stations": "Pittendreigh City"
+ },
+ {
+ "name": "Hmon",
+ "pos_x": -18.65625,
+ "pos_y": 101.875,
+ "pos_z": 114.46875,
+ "stations": "Gann Vision,Harding Enterprise,Lambert Vision,Nordenskiold Arsenal,Silves Works"
+ },
+ {
+ "name": "Hmona",
+ "pos_x": -39.625,
+ "pos_y": 133.5625,
+ "pos_z": 24.46875,
+ "stations": "Skvortsov Refinery,Mills Terminal"
+ },
+ {
+ "name": "Hmontabozho",
+ "pos_x": 42.21875,
+ "pos_y": 91.375,
+ "pos_z": 102.78125,
+ "stations": "Euler Orbital"
+ },
+ {
+ "name": "HN Bootis",
+ "pos_x": -1.53125,
+ "pos_y": 46.21875,
+ "pos_z": 27.46875,
+ "stations": "Duckworth Orbital"
+ },
+ {
+ "name": "Hnossit",
+ "pos_x": 66.75,
+ "pos_y": -32.78125,
+ "pos_z": -80.15625,
+ "stations": "Danvers Retreat,Ore Platform,Pellegrino Depot"
+ },
+ {
+ "name": "HO Bootis",
+ "pos_x": -23.125,
+ "pos_y": 73.84375,
+ "pos_z": 27.40625,
+ "stations": "Ore Platform,Altshuller Port"
+ },
+ {
+ "name": "Ho Hsi",
+ "pos_x": -0.4375,
+ "pos_y": -11.46875,
+ "pos_z": 61.28125,
+ "stations": "Hand Ring,Steiner Hub,Alexeyev Enterprise,Smith's Progress,Fairbairn Keep"
+ },
+ {
+ "name": "Ho Hsien",
+ "pos_x": -76.03125,
+ "pos_y": 21.90625,
+ "pos_z": 38.09375,
+ "stations": "Dutton Station,Bushnell Enterprise,Onufriyenko Hub"
+ },
+ {
+ "name": "Ho Panang",
+ "pos_x": 84.6875,
+ "pos_y": 79.59375,
+ "pos_z": -116.59375,
+ "stations": "Still Mine,Haipeng Settlement,Morrison Plant"
+ },
+ {
+ "name": "Ho Pani",
+ "pos_x": 169.09375,
+ "pos_y": -150.5625,
+ "pos_z": 74.1875,
+ "stations": "Zwicky Vision"
+ },
+ {
+ "name": "Ho Parerme",
+ "pos_x": 62.21875,
+ "pos_y": -0.71875,
+ "pos_z": -30.15625,
+ "stations": "Brothers Hub,Finney City,Haldeman II Vision,Elgin Beacon,Morgan Dock"
+ },
+ {
+ "name": "Ho Pares",
+ "pos_x": 126.21875,
+ "pos_y": -105.15625,
+ "pos_z": 133.4375,
+ "stations": "Ferguson Mines,Coande Vision,Beg Settlement"
+ },
+ {
+ "name": "Hodack",
+ "pos_x": 60.28125,
+ "pos_y": 23.53125,
+ "pos_z": 46.40625,
+ "stations": "Evangelisti Settlement,Chilton Vista"
+ },
+ {
+ "name": "Hoder",
+ "pos_x": -21.1875,
+ "pos_y": -5.25,
+ "pos_z": -75.3125,
+ "stations": "Jacquard Orbital,Sullivan Dock"
+ },
+ {
+ "name": "Hoduma",
+ "pos_x": -125.65625,
+ "pos_y": 90.09375,
+ "pos_z": -57.53125,
+ "stations": "Euthymenes Landing,Thesiger Landing,Fast Terminal,Shirley Laboratory"
+ },
+ {
+ "name": "Hodunin",
+ "pos_x": 36.875,
+ "pos_y": 140.53125,
+ "pos_z": -2.84375,
+ "stations": "Andrews Orbital,Davy Forum,Mackenzie Port,Temple Station"
+ },
+ {
+ "name": "Hodur",
+ "pos_x": 140.78125,
+ "pos_y": -20.96875,
+ "pos_z": -75,
+ "stations": "al-Khowarizmi Station"
+ },
+ {
+ "name": "Hodurngana",
+ "pos_x": -120.21875,
+ "pos_y": -7.875,
+ "pos_z": -103.09375,
+ "stations": "Kimberlin Port,Vasilyev Relay"
+ },
+ {
+ "name": "Hofada",
+ "pos_x": 90.84375,
+ "pos_y": 35.34375,
+ "pos_z": 38.625,
+ "stations": "Tiptree Beacon"
+ },
+ {
+ "name": "Hoff",
+ "pos_x": -9.9375,
+ "pos_y": -69.21875,
+ "pos_z": -72.9375,
+ "stations": "Leclerc Terminal,Vesalius Terminal,Oluwafemi Terminal,Abe Camp,Tognini Station,Lundwall Hub,Clairaut Survey"
+ },
+ {
+ "name": "Hohnpeiadja",
+ "pos_x": -100.875,
+ "pos_y": 7.875,
+ "pos_z": -132.6875,
+ "stations": "Shawl Camp"
+ },
+ {
+ "name": "Hohnpet",
+ "pos_x": -74.6875,
+ "pos_y": -150.34375,
+ "pos_z": 46.8125,
+ "stations": "Smirnova Hub,Rosse Orbital,McCrea Horizons"
+ },
+ {
+ "name": "Hohnpete",
+ "pos_x": -94.46875,
+ "pos_y": 54.4375,
+ "pos_z": 140.84375,
+ "stations": "Garn Point"
+ },
+ {
+ "name": "Hoji",
+ "pos_x": 134.28125,
+ "pos_y": -104.1875,
+ "pos_z": 25.90625,
+ "stations": "Watanabe Beacon,Fowler Station,Siegel Station,Helin Observatory,Fabricius Ring,Riazuddin Reach,Messerschmitt Vision,Arkhangelsky Arsenal,Webb Stop"
+ },
+ {
+ "name": "Hojin",
+ "pos_x": 102.3125,
+ "pos_y": -171.21875,
+ "pos_z": 106.78125,
+ "stations": "Hughes Station,Fedden Landing,Pellegrino Vision"
+ },
+ {
+ "name": "Hoko",
+ "pos_x": -26.84375,
+ "pos_y": -32.5,
+ "pos_z": -4.34375,
+ "stations": "Onufrienko City,Schade Silo,Mach Horizons,Goeppert-Mayer Port,Vesalius Hub"
+ },
+ {
+ "name": "Holda",
+ "pos_x": 46.34375,
+ "pos_y": 100.4375,
+ "pos_z": 59.40625,
+ "stations": "Swigert Stop,Kraepelin Reach"
+ },
+ {
+ "name": "Holiacan",
+ "pos_x": -51.25,
+ "pos_y": 67.34375,
+ "pos_z": -21.46875,
+ "stations": "Hopi,Mike Tapa Astronautics Ltd,al-Khayyam Holdings,Fortress Yarrow,Schmidt Gateway"
+ },
+ {
+ "name": "Hollatja",
+ "pos_x": 25.3125,
+ "pos_y": -32.15625,
+ "pos_z": 35.8125,
+ "stations": "Popper Keep,Fermat City"
+ },
+ {
+ "name": "Holler",
+ "pos_x": -93.3125,
+ "pos_y": -75.625,
+ "pos_z": 114.09375,
+ "stations": "Hume Ring,Chebyshev Enterprise,Bunch Port,Macan Relay,Hertz's Inheritance"
+ },
+ {
+ "name": "Hollobrigo",
+ "pos_x": 99.84375,
+ "pos_y": -126.21875,
+ "pos_z": -46.28125,
+ "stations": "Ludwig Struve City,Rigaux Vision,Wenzel Port,Green Terminal,Shea Gateway"
+ },
+ {
+ "name": "Hollos",
+ "pos_x": 95.78125,
+ "pos_y": -92.84375,
+ "pos_z": 68.1875,
+ "stations": "Fowler Vision,Barcelona Vision,Pond Port,Bingzhen Hub,Bauschinger Landing,Cuffey Vision,Beer Terminal,Bharadwaj Hub,Poyser Terminal,Whittle Prospect"
+ },
+ {
+ "name": "Hollu",
+ "pos_x": 31.125,
+ "pos_y": -17.8125,
+ "pos_z": -118.3125,
+ "stations": "Creighton Base,Macan Terminal"
+ },
+ {
+ "name": "Holo",
+ "pos_x": 20.84375,
+ "pos_y": -9.6875,
+ "pos_z": -110.875,
+ "stations": "Thomas Gateway,Greenleaf Barracks,Lysenko Station,Kafka Observatory"
+ },
+ {
+ "name": "Holocan",
+ "pos_x": -70.1875,
+ "pos_y": -94.28125,
+ "pos_z": 74.9375,
+ "stations": "Ahern Gateway,Merchiston Station"
+ },
+ {
+ "name": "Holva",
+ "pos_x": 58.6875,
+ "pos_y": -170.96875,
+ "pos_z": -41.96875,
+ "stations": "Kreutz Orbital"
+ },
+ {
+ "name": "Holvait",
+ "pos_x": -86.34375,
+ "pos_y": 9,
+ "pos_z": -91.125,
+ "stations": "Nikolayev Hangar"
+ },
+ {
+ "name": "Holvandalla",
+ "pos_x": -131.875,
+ "pos_y": -68.75,
+ "pos_z": -14.59375,
+ "stations": "Shalatula Ring,Abel Barracks,Reynolds' Inheritance,Asher Dock,Farrukh Dock"
+ },
+ {
+ "name": "Hong",
+ "pos_x": -1.09375,
+ "pos_y": -102.625,
+ "pos_z": 35.3125,
+ "stations": "Kempf Port"
+ },
+ {
+ "name": "Hongbiri",
+ "pos_x": -115.53125,
+ "pos_y": 1.9375,
+ "pos_z": -97.40625,
+ "stations": "Schiltberger Beacon,Shirley Enterprise"
+ },
+ {
+ "name": "Honges",
+ "pos_x": -84.125,
+ "pos_y": -65.125,
+ "pos_z": 3.96875,
+ "stations": "Cabot Gateway,Schiltberger Station,Phillpotts Port,Forsskal Settlement,al-Khayyam Enterprise"
+ },
+ {
+ "name": "Honggoro",
+ "pos_x": 87.84375,
+ "pos_y": -213.46875,
+ "pos_z": 84.21875,
+ "stations": "Keeler Point"
+ },
+ {
+ "name": "Honii",
+ "pos_x": -43.125,
+ "pos_y": 80.28125,
+ "pos_z": -61.28125,
+ "stations": "Lovelace Installation,Borman Installation,Sacco Terminal,England Plant"
+ },
+ {
+ "name": "Honir",
+ "pos_x": -15.3125,
+ "pos_y": -145.84375,
+ "pos_z": 132.0625,
+ "stations": "Almagro Survey,Maunder Station,Suydam Works"
+ },
+ {
+ "name": "Honiu",
+ "pos_x": 17.71875,
+ "pos_y": -91.5625,
+ "pos_z": 105.65625,
+ "stations": "Biesbroeck Terminal"
+ },
+ {
+ "name": "Honoss",
+ "pos_x": 37.1875,
+ "pos_y": -153.71875,
+ "pos_z": 136.84375,
+ "stations": "Al-Khujandi Camp"
+ },
+ {
+ "name": "Honoto",
+ "pos_x": 75.65625,
+ "pos_y": 11.4375,
+ "pos_z": 38.46875,
+ "stations": "Gagarin Landing,Kennedy Survey,Jones Relay"
+ },
+ {
+ "name": "Hoora Ating",
+ "pos_x": -0.5,
+ "pos_y": -94.6875,
+ "pos_z": 89.21875,
+ "stations": "Addams Prospect,Hawker Platform,Legendre Settlement"
+ },
+ {
+ "name": "Hoorajo",
+ "pos_x": -112.5625,
+ "pos_y": -69.40625,
+ "pos_z": -45.9375,
+ "stations": "Gillekens Prospect"
+ },
+ {
+ "name": "Hooriayan",
+ "pos_x": -23.34375,
+ "pos_y": 78,
+ "pos_z": 18.125,
+ "stations": "Davis Port"
+ },
+ {
+ "name": "Hopian",
+ "pos_x": -72.375,
+ "pos_y": 133.4375,
+ "pos_z": -37.90625,
+ "stations": "Caidin Settlement,Baydukov's Claim"
+ },
+ {
+ "name": "Horae",
+ "pos_x": -1.8125,
+ "pos_y": -57.65625,
+ "pos_z": 8.1875,
+ "stations": "McDivitt Dock,Haber Hub"
+ },
+ {
+ "name": "Horagalles",
+ "pos_x": -11.5625,
+ "pos_y": -35.3125,
+ "pos_z": 51.71875,
+ "stations": "Mallory Landing,Plexico Orbital,Matheson Prospect"
+ },
+ {
+ "name": "Hors",
+ "pos_x": 37.1875,
+ "pos_y": -34.71875,
+ "pos_z": 25.84375,
+ "stations": "Candy Cavern,Tuan Point"
+ },
+ {
+ "name": "Hosakab",
+ "pos_x": -59.96875,
+ "pos_y": -83.625,
+ "pos_z": -117.59375,
+ "stations": "Kanwar Beacon"
+ },
+ {
+ "name": "Hosan",
+ "pos_x": -152.65625,
+ "pos_y": -82.3125,
+ "pos_z": -9.34375,
+ "stations": "Poncelet Plant"
+ },
+ {
+ "name": "Hosuets",
+ "pos_x": -118.84375,
+ "pos_y": -22.21875,
+ "pos_z": 105.25,
+ "stations": "Feynman Vision,Popper Settlement"
+ },
+ {
+ "name": "Hosuetta",
+ "pos_x": -27.40625,
+ "pos_y": 74.1875,
+ "pos_z": 144.9375,
+ "stations": "Bursch Orbital,Forrester Terminal,Solovyov Enterprise,Brongniart Port"
+ },
+ {
+ "name": "Hosus",
+ "pos_x": 139.09375,
+ "pos_y": -8.21875,
+ "pos_z": -37.96875,
+ "stations": "Gordon Colony,Grunsfeld Orbital"
+ },
+ {
+ "name": "Hotadital",
+ "pos_x": 3.0625,
+ "pos_y": -22.15625,
+ "pos_z": -142.96875,
+ "stations": "Wilcutt Base"
+ },
+ {
+ "name": "Hotai",
+ "pos_x": 133.78125,
+ "pos_y": 93.5625,
+ "pos_z": -37.71875,
+ "stations": "Usachov's Claim,Davis Base"
+ },
+ {
+ "name": "Hotaiko",
+ "pos_x": 133.375,
+ "pos_y": 6.40625,
+ "pos_z": -7.53125,
+ "stations": "Ore Orbital,Dirichlet Enterprise"
+ },
+ {
+ "name": "Hotototo",
+ "pos_x": 86.0625,
+ "pos_y": -138.4375,
+ "pos_z": 102.1875,
+ "stations": "Paola Dock,Baracchi's Folly,Husband Station"
+ },
+ {
+ "name": "Hou Chema",
+ "pos_x": 137.0625,
+ "pos_y": 69.21875,
+ "pos_z": 41.59375,
+ "stations": "Lukyanenko Mine"
+ },
+ {
+ "name": "Hou Hsien",
+ "pos_x": -38.0625,
+ "pos_y": 99.5,
+ "pos_z": -30.375,
+ "stations": "Hill Hub,Lukyanenko Arsenal"
+ },
+ {
+ "name": "Hou Main",
+ "pos_x": -35.15625,
+ "pos_y": 69.34375,
+ "pos_z": -81.96875,
+ "stations": "Schade Prospect,Guin Prospect,Hudson Holdings"
+ },
+ {
+ "name": "Hou Mara",
+ "pos_x": -142.3125,
+ "pos_y": 36.0625,
+ "pos_z": 79.21875,
+ "stations": "Luiken Barracks,Oppenheimer Orbital,Wolf Dock,Zebrowski Camp"
+ },
+ {
+ "name": "Hou Mayo",
+ "pos_x": 105.59375,
+ "pos_y": -107.5,
+ "pos_z": -25.0625,
+ "stations": "Saunders Beacon,Mouhot Relay,Dingle Penal colony,Kaneda City"
+ },
+ {
+ "name": "Hou Tuba",
+ "pos_x": -62.75,
+ "pos_y": -58.5625,
+ "pos_z": 122.03125,
+ "stations": "Lamarck Hub,Feoktistov Terminal,Henson's Inheritance,Moore's Folly"
+ },
+ {
+ "name": "Hou Warwa",
+ "pos_x": 9.5,
+ "pos_y": -0.46875,
+ "pos_z": -103.875,
+ "stations": "Joliot-Curie Gateway"
+ },
+ {
+ "name": "Hou Yangk",
+ "pos_x": 86.8125,
+ "pos_y": -8.28125,
+ "pos_z": 127.59375,
+ "stations": "Bunsen Port,Napier Port,Shuttleworth Dock,Piccard Penal colony"
+ },
+ {
+ "name": "Hou Zi",
+ "pos_x": 48.34375,
+ "pos_y": -235.25,
+ "pos_z": 35.875,
+ "stations": "Fieseler Outpost"
+ },
+ {
+ "name": "Hou Zu",
+ "pos_x": 49.125,
+ "pos_y": 39.3125,
+ "pos_z": -101.09375,
+ "stations": "Precourt City,Piaget City,Russell's Inheritance,Pawelczyk Orbital,Weyl Installation"
+ },
+ {
+ "name": "Howard",
+ "pos_x": -71.21875,
+ "pos_y": -15.75,
+ "pos_z": -32.875,
+ "stations": "Rogatino High,Messerschmid Bastion,Serling Station"
+ },
+ {
+ "name": "HP Cancri",
+ "pos_x": 41.9375,
+ "pos_y": 38.28125,
+ "pos_z": -49.5,
+ "stations": "Archambault Hangar,Massimino Arsenal,Gooch Station"
+ },
+ {
+ "name": "HR 1013",
+ "pos_x": 58.75,
+ "pos_y": -135.59375,
+ "pos_z": -68.625,
+ "stations": "Hunt Base,Kojima Hangar"
+ },
+ {
+ "name": "HR 1014",
+ "pos_x": 124.3125,
+ "pos_y": -125.53125,
+ "pos_z": 30.03125,
+ "stations": "Sitter Orbital,Sugie's Progress,Bowell Hub,Gustafsson Dock"
+ },
+ {
+ "name": "HR 1064",
+ "pos_x": 91.53125,
+ "pos_y": -86.5625,
+ "pos_z": 25.3125,
+ "stations": "Lalande Bastion,Clark Terminal,McKean City,Piccard Settlement,Verrazzano Forum,Nakano Market,Kobayashi Port,Somayaji Port,Thomas Port,Baxter Landing"
+ },
+ {
+ "name": "HR 1075",
+ "pos_x": 116.59375,
+ "pos_y": -173,
+ "pos_z": -45.21875,
+ "stations": "Lambert Station,Whipple Terminal,Payne-Scott Dock,Yerka Vision,Stein Settlement,Pannekoek Stop,Meredith Lab,Tomita Depot,Pond Terminal"
+ },
+ {
+ "name": "HR 1076",
+ "pos_x": 60.59375,
+ "pos_y": -91.9375,
+ "pos_z": -26.15625,
+ "stations": "Elwood Dock"
+ },
+ {
+ "name": "HR 108",
+ "pos_x": -15.53125,
+ "pos_y": -103.875,
+ "pos_z": 0.46875,
+ "stations": "Rond d'Alembert Enterprise,Cook Base,Terry Gateway,Krylov City,Anvil Point"
+ },
+ {
+ "name": "HR 1124",
+ "pos_x": -116.875,
+ "pos_y": 32.125,
+ "pos_z": -134.1875,
+ "stations": "Webb Reach"
+ },
+ {
+ "name": "HR 1139",
+ "pos_x": 31.875,
+ "pos_y": -99.03125,
+ "pos_z": -91.28125,
+ "stations": "Nasmyth Gateway,Merbold Terminal,Slade Base"
+ },
+ {
+ "name": "HR 115",
+ "pos_x": -31.34375,
+ "pos_y": -135.71875,
+ "pos_z": -6.96875,
+ "stations": "Keeler Reach,Schmidt Beacon"
+ },
+ {
+ "name": "HR 1168",
+ "pos_x": 164.6875,
+ "pos_y": -185.78125,
+ "pos_z": -13.0625,
+ "stations": "Celsius Prospect,Paczynski Terminal,Michael's Folly"
+ },
+ {
+ "name": "HR 1179",
+ "pos_x": 57.65625,
+ "pos_y": -96.59375,
+ "pos_z": -52.6875,
+ "stations": "Giles Hub,Meredith Terminal,Friedman Base"
+ },
+ {
+ "name": "HR 1183",
+ "pos_x": -72.875,
+ "pos_y": -145.09375,
+ "pos_z": -336.96875,
+ "stations": "Arc's Faith"
+ },
+ {
+ "name": "HR 1185",
+ "pos_x": -64.65625,
+ "pos_y": -148.9375,
+ "pos_z": -330.40625,
+ "stations": "Ceres Tarn,The Indra"
+ },
+ {
+ "name": "HR 1201",
+ "pos_x": -13.9375,
+ "pos_y": -60.84375,
+ "pos_z": -119.5,
+ "stations": "Antonelli Station,Grego Base,Emshwiller Penal colony"
+ },
+ {
+ "name": "HR 1233",
+ "pos_x": 0.875,
+ "pos_y": -66.03125,
+ "pos_z": -112.65625,
+ "stations": "Haise Platform,Shiras' Inheritance,Kopra Depot"
+ },
+ {
+ "name": "HR 1245",
+ "pos_x": 140.375,
+ "pos_y": -142.6875,
+ "pos_z": -4.34375,
+ "stations": "Whelan Dock,Mozhaysky Ring,Crown Dock,Flamsteed Ring,Montanari Dock,Vavrova Terminal,Michael Orbital,Payne-Scott Dock,Korolev Gateway,Wallis Relay,Cousteau Prospect"
+ },
+ {
+ "name": "HR 1254",
+ "pos_x": 5.34375,
+ "pos_y": -58.4375,
+ "pos_z": -97.375,
+ "stations": "McDonald Prospect,Ivanishin City,Klimuk City,Ellis Orbital,Potrykus Market,Linteris Enterprise,Bhabha Hub,Matheson Hub"
+ },
+ {
+ "name": "HR 1257",
+ "pos_x": 13.65625,
+ "pos_y": -64.84375,
+ "pos_z": -94.5625,
+ "stations": "Bond Station,Jacobi Platform,Miller Settlement,Lessing Depot"
+ },
+ {
+ "name": "HR 1279",
+ "pos_x": -5,
+ "pos_y": -61.8125,
+ "pos_z": -128.125,
+ "stations": "Hubble Dock,Coelho Camp,Chretien Dock,Bosch Enterprise,Larson Hub"
+ },
+ {
+ "name": "HR 1354",
+ "pos_x": -7.71875,
+ "pos_y": -56.71875,
+ "pos_z": -146.84375,
+ "stations": "Olsen Colony,Thorne's Progress"
+ },
+ {
+ "name": "HR 1364",
+ "pos_x": 166.71875,
+ "pos_y": -178.375,
+ "pos_z": -62.65625,
+ "stations": "Roth Vision,Sugie Installation"
+ },
+ {
+ "name": "HR 1385",
+ "pos_x": -6.78125,
+ "pos_y": -56.59375,
+ "pos_z": -154.65625,
+ "stations": "Liska Dock,Avdeyev Installation,McDivitt Outpost"
+ },
+ {
+ "name": "HR 1403",
+ "pos_x": -10.46875,
+ "pos_y": -47.15625,
+ "pos_z": -145.9375,
+ "stations": "Chang-Diaz Station,Zudov Colony,Hopkins Orbital,Euthymenes Base,Caidin Base"
+ },
+ {
+ "name": "HR 1436",
+ "pos_x": 22.96875,
+ "pos_y": -64.40625,
+ "pos_z": -124.34375,
+ "stations": "Camarda Outpost,Higginbotham Plant,Manarov Dock,Arnarson Keep"
+ },
+ {
+ "name": "HR 1475",
+ "pos_x": 113.46875,
+ "pos_y": -93.5625,
+ "pos_z": 7.09375,
+ "stations": "Ortiz Moreno City,Fiorilla Refinery,Leuschner City,Clairaut Vista,Hollander Vision,Youll Terminal,Brundage Port,McCrea Hub,Backlund Dock,Levi-Civita Keep,Bessel City,Tully Vision,Michell Prospect,Semeonis Point,Barnes Beacon"
+ },
+ {
+ "name": "HR 148",
+ "pos_x": 84.96875,
+ "pos_y": -204.71875,
+ "pos_z": 64.46875,
+ "stations": "Burns Vision,Ahnert-Rohlfs Ring,Baillaud Orbital,Bassford's Folly,Mitchell Depot"
+ },
+ {
+ "name": "HR 1480",
+ "pos_x": 23.6875,
+ "pos_y": -65.625,
+ "pos_z": -144.40625,
+ "stations": "Worlidge Landing,Hornblower Mines,Helms Installation"
+ },
+ {
+ "name": "HR 1487",
+ "pos_x": 84.25,
+ "pos_y": -112.84375,
+ "pos_z": -136.53125,
+ "stations": "Artemis' Nest"
+ },
+ {
+ "name": "HR 1491",
+ "pos_x": -100.5,
+ "pos_y": 51.9375,
+ "pos_z": -103.75,
+ "stations": "Herjulfsson Settlement,Jordan Mines"
+ },
+ {
+ "name": "HR 1507",
+ "pos_x": 17.5625,
+ "pos_y": -54.0625,
+ "pos_z": -138.28125,
+ "stations": "Budrys Beacon,Rolland Platform"
+ },
+ {
+ "name": "HR 1593",
+ "pos_x": -58.1875,
+ "pos_y": 24.25,
+ "pos_z": -97.53125,
+ "stations": "Goddard Port,Conway Station,McDaniel Horizons,Schmitt Orbital,Akers City,Kononenko Enterprise,Nobel Bastion,Banach's Progress"
+ },
+ {
+ "name": "HR 1594",
+ "pos_x": -50.78125,
+ "pos_y": 24.15625,
+ "pos_z": -71,
+ "stations": "Lowry Works,Chilton Port,Trinh Gateway,Roberts Settlement"
+ },
+ {
+ "name": "HR 1597",
+ "pos_x": 78.1875,
+ "pos_y": -60.875,
+ "pos_z": -3.4375,
+ "stations": "Jean City,Elst Prospect,Lilienthal Installation,De Keep"
+ },
+ {
+ "name": "HR 1613",
+ "pos_x": 54.3125,
+ "pos_y": -68.0625,
+ "pos_z": -136.375,
+ "stations": "Thornycroft Platform,von Bellingshausen Mines,Bixby Prospect"
+ },
+ {
+ "name": "HR 1621",
+ "pos_x": 108.375,
+ "pos_y": -105.90625,
+ "pos_z": -127.34375,
+ "stations": "Poisson Mine,Robinson Relay"
+ },
+ {
+ "name": "HR 1645",
+ "pos_x": 113.1875,
+ "pos_y": -104,
+ "pos_z": -111.25,
+ "stations": "Folland Vision,Zarnecki Port,Paez Prospect"
+ },
+ {
+ "name": "HR 17",
+ "pos_x": -87.40625,
+ "pos_y": -45.1875,
+ "pos_z": -39.03125,
+ "stations": "Schrodinger City,Duke Hub,Mayr Enterprise,Compton Laboratory,Armstrong Barracks"
+ },
+ {
+ "name": "HR 1717",
+ "pos_x": 55.75,
+ "pos_y": -55.71875,
+ "pos_z": -131.125,
+ "stations": "Martins Dock,Ali Escape,Griffith Settlement,Fernao do Po's Inheritance,Jones Dock"
+ },
+ {
+ "name": "HR 1737",
+ "pos_x": 82.5625,
+ "pos_y": -70.78125,
+ "pos_z": -117.21875,
+ "stations": "Madsen Park,Yolen Station,Dias Horizons,Dirichlet Enterprise,Stableford Enterprise"
+ },
+ {
+ "name": "HR 1782",
+ "pos_x": 68.78125,
+ "pos_y": -59.84375,
+ "pos_z": -157.1875,
+ "stations": "Milnor Dock,Tavares Works,Lunan Settlement,Payson Enterprise"
+ },
+ {
+ "name": "HR 1785",
+ "pos_x": 107.78125,
+ "pos_y": -81,
+ "pos_z": -91.59375,
+ "stations": "Brooks Dock,Foster Base,Smeaton Base"
+ },
+ {
+ "name": "HR 1812",
+ "pos_x": 88.34375,
+ "pos_y": -66.28125,
+ "pos_z": -96.8125,
+ "stations": "McMullen Vision"
+ },
+ {
+ "name": "HR 1856",
+ "pos_x": 115.96875,
+ "pos_y": -77.875,
+ "pos_z": -34.71875,
+ "stations": "Fischer Installation,Schwarzschild Prospect,Royo Hangar,Godwin Depot,Salgari Terminal"
+ },
+ {
+ "name": "HR 1877",
+ "pos_x": 132.3125,
+ "pos_y": -88.4375,
+ "pos_z": -66.03125,
+ "stations": "Poncelet Terminal,Egan Survey"
+ },
+ {
+ "name": "HR 1915",
+ "pos_x": 121.5,
+ "pos_y": -79.96875,
+ "pos_z": -92.1875,
+ "stations": "Fraas Settlement,Bethke Point"
+ },
+ {
+ "name": "HR 1940",
+ "pos_x": 62.46875,
+ "pos_y": -40.71875,
+ "pos_z": -117.125,
+ "stations": "Frobenius Vision,Nikitin Mines,Bulychev Penitentiary"
+ },
+ {
+ "name": "HR 1955",
+ "pos_x": 63.125,
+ "pos_y": -40.125,
+ "pos_z": -137.3125,
+ "stations": "Serling Installation,Nicolet Colony"
+ },
+ {
+ "name": "HR 197",
+ "pos_x": -7.71875,
+ "pos_y": -86.84375,
+ "pos_z": -3.21875,
+ "stations": "Fourier Prospect,Jeschke Refinery,Fernandez Vision"
+ },
+ {
+ "name": "HR 1978",
+ "pos_x": 60,
+ "pos_y": -34.9375,
+ "pos_z": -149.5,
+ "stations": "Morin Enterprise,Clerk Base,Clark Terminal"
+ },
+ {
+ "name": "HR 198",
+ "pos_x": 44.90625,
+ "pos_y": -202.59375,
+ "pos_z": 32.40625,
+ "stations": "Mukai Landing,Hogg Beacon"
+ },
+ {
+ "name": "HR 1980",
+ "pos_x": 53.875,
+ "pos_y": -32.5,
+ "pos_z": -54.625,
+ "stations": "Foale Orbital,Sladek Enterprise,Low City,Galois Barracks,Pavlou Landing"
+ },
+ {
+ "name": "HR 1981",
+ "pos_x": 136.375,
+ "pos_y": -83.59375,
+ "pos_z": -63.59375,
+ "stations": "Amedeo Plana Dock,Foden Settlement"
+ },
+ {
+ "name": "HR 2204",
+ "pos_x": 168.21875,
+ "pos_y": -86.71875,
+ "pos_z": -37.8125,
+ "stations": "Baliunas Arsenal,Struzan Stop"
+ },
+ {
+ "name": "HR 2209",
+ "pos_x": -91.6875,
+ "pos_y": 68.125,
+ "pos_z": -132.59375,
+ "stations": "Bethe Refinery,Szebehely Landing,Lefschetz Vision,Howard Hub"
+ },
+ {
+ "name": "HR 2265",
+ "pos_x": 169.125,
+ "pos_y": -76.03125,
+ "pos_z": -80,
+ "stations": "Slonczewski Oasis"
+ },
+ {
+ "name": "HR 2283",
+ "pos_x": 87.9375,
+ "pos_y": -49,
+ "pos_z": 19.15625,
+ "stations": "Hobaugh Terminal,Musgrave Dock,Euler Hub,Wilcutt Hub"
+ },
+ {
+ "name": "HR 2365",
+ "pos_x": -66.53125,
+ "pos_y": 50.375,
+ "pos_z": -82.4375,
+ "stations": "Lichtenberg Station,Maybury Depot,Lysenko Ring,Fossey Station"
+ },
+ {
+ "name": "HR 2439",
+ "pos_x": 18.1875,
+ "pos_y": 16.90625,
+ "pos_z": -104,
+ "stations": "Pawelczyk City"
+ },
+ {
+ "name": "HR 244",
+ "pos_x": -51,
+ "pos_y": -1.59375,
+ "pos_z": -33.75,
+ "stations": "Garneau Port,Kepler City,Hart Port,Malzberg Laboratory,Fuglesang Hub,Malyshev Colony"
+ },
+ {
+ "name": "HR 2551",
+ "pos_x": 88.71875,
+ "pos_y": 16.59375,
+ "pos_z": -182.09375,
+ "stations": "Noon Beacon,Chebyshev Reach"
+ },
+ {
+ "name": "HR 2622",
+ "pos_x": 59.5,
+ "pos_y": 0.03125,
+ "pos_z": -73.34375,
+ "stations": "Pascal Horizons,Wingrove Installation"
+ },
+ {
+ "name": "HR 2711",
+ "pos_x": 24.46875,
+ "pos_y": 40.5,
+ "pos_z": -130.25,
+ "stations": "Wilmore Station,Cabrera Hub,Khan Station,Ray Enterprise,Babcock Orbital"
+ },
+ {
+ "name": "HR 2776",
+ "pos_x": -12.8125,
+ "pos_y": 47.96875,
+ "pos_z": -104.5625,
+ "stations": "Sharma Holdings,Dobrovolski Platform,Herjulfsson Prospect"
+ },
+ {
+ "name": "HR 2798",
+ "pos_x": 83.0625,
+ "pos_y": 6.15625,
+ "pos_z": -84.25,
+ "stations": "Cugnot Station,Gardner Hub"
+ },
+ {
+ "name": "HR 2848",
+ "pos_x": 99.03125,
+ "pos_y": -59.0625,
+ "pos_z": 56.375,
+ "stations": "Zander Hub"
+ },
+ {
+ "name": "HR 2883",
+ "pos_x": 65.0625,
+ "pos_y": 8.4375,
+ "pos_z": -63.125,
+ "stations": "Barth Holdings,Dutton Gateway,Neumann Enterprise,White Station,Satcher Plant"
+ },
+ {
+ "name": "HR 29",
+ "pos_x": -76.4375,
+ "pos_y": -173.78125,
+ "pos_z": -11.71875,
+ "stations": "Artin's Pride,Laumer Orbital"
+ },
+ {
+ "name": "HR 2906",
+ "pos_x": 69.8125,
+ "pos_y": -1.1875,
+ "pos_z": -43.9375,
+ "stations": "Erdos Terminal,Heyerdahl Laboratory,Baird Penal colony,Alvarez de Pineda City,Steakley Orbital"
+ },
+ {
+ "name": "HR 2926",
+ "pos_x": 35.09375,
+ "pos_y": 50.3125,
+ "pos_z": -124.6875,
+ "stations": "Walz Enterprise,Underwood Hub,Engle Gateway,Szilard Terminal,Usachov Port,Battuta Hub,Nespoli Terminal"
+ },
+ {
+ "name": "HR 299",
+ "pos_x": 70.59375,
+ "pos_y": -225.1875,
+ "pos_z": 34.03125,
+ "stations": "Agrippa Orbital,Lloyd Wright Enterprise"
+ },
+ {
+ "name": "HR 3007",
+ "pos_x": 131.71875,
+ "pos_y": -14,
+ "pos_z": -45.625,
+ "stations": "Reed's Inheritance,Grechko Station,Trevithick Plant"
+ },
+ {
+ "name": "HR 3072",
+ "pos_x": 90.3125,
+ "pos_y": 25.8125,
+ "pos_z": -89.1875,
+ "stations": "Altshuller Terminal,Burgess Landing"
+ },
+ {
+ "name": "HR 3193",
+ "pos_x": 5.90625,
+ "pos_y": 83.21875,
+ "pos_z": -132.53125,
+ "stations": "Matteucci Mines,Sinclair Settlement,Bowen Hub,Cooper Keep,Qian Beacon"
+ },
+ {
+ "name": "HR 32",
+ "pos_x": 132.5,
+ "pos_y": -159.25,
+ "pos_z": 99.1875,
+ "stations": "The Heraklion"
+ },
+ {
+ "name": "HR 325",
+ "pos_x": -2.0625,
+ "pos_y": -223.375,
+ "pos_z": -20.75,
+ "stations": "Eisinga Ring,Proctor Station,Ore Beacon,Farouk Base,Petlyakov Ring"
+ },
+ {
+ "name": "HR 3277",
+ "pos_x": -33.71875,
+ "pos_y": 95.75,
+ "pos_z": -129.40625,
+ "stations": "Norman Enterprise,Mitchison Installation,Witt Laboratory"
+ },
+ {
+ "name": "HR 3316",
+ "pos_x": 100.71875,
+ "pos_y": 19.8125,
+ "pos_z": -51.125,
+ "stations": "Schilling Orbital,Carleson Oasis,Sylvester Plant"
+ },
+ {
+ "name": "HR 3495",
+ "pos_x": 181.78125,
+ "pos_y": -46.71875,
+ "pos_z": 36.3125,
+ "stations": "Albategnius Installation,Lewis Settlement"
+ },
+ {
+ "name": "HR 3499",
+ "pos_x": 13.59375,
+ "pos_y": 58.0625,
+ "pos_z": -70.6875,
+ "stations": "Goddard Hub,Antonelli Prospect,Wohler City,Molina Station,Bentham Settlement"
+ },
+ {
+ "name": "HR 358",
+ "pos_x": 25.1875,
+ "pos_y": -252.78125,
+ "pos_z": -10.15625,
+ "stations": "Pittendreigh Stop,Lindstrand Station,Kingsmill Holdings"
+ },
+ {
+ "name": "HR 3584",
+ "pos_x": 112,
+ "pos_y": 38.53125,
+ "pos_z": -48.65625,
+ "stations": "Gooch Orbital"
+ },
+ {
+ "name": "HR 3635",
+ "pos_x": 64.0625,
+ "pos_y": 75.34375,
+ "pos_z": -79.59375,
+ "stations": "Walker Terminal,Kozeyev Orbital,Brahe Terminal,Kelly Dock,Volynov Hub,Temple Palace,Scalzi Depot,Phillips' Inheritance,Sekowski Depot"
+ },
+ {
+ "name": "HR 3714",
+ "pos_x": 136.84375,
+ "pos_y": 65.34375,
+ "pos_z": -58.15625,
+ "stations": "Bobko Terminal,Dutton Enterprise,Musa al-Khwarizmi Enterprise"
+ },
+ {
+ "name": "HR 3747",
+ "pos_x": -33.0625,
+ "pos_y": 96.34375,
+ "pos_z": -91.75,
+ "stations": "Kotzebue Orbital,Soto Hub,McAuley Dock,Dirichlet Enterprise,Pytheas Orbital,Napier Hub,Smith Enterprise,Baker Beacon,Peano Observatory,Barcelos Beacon,Beaumont Base,Back Vista"
+ },
+ {
+ "name": "HR 3762",
+ "pos_x": 130.40625,
+ "pos_y": 97.375,
+ "pos_z": -80.34375,
+ "stations": "Cooper Port,Comino Hub"
+ },
+ {
+ "name": "HR 394",
+ "pos_x": -8.53125,
+ "pos_y": -196.6875,
+ "pos_z": -38.46875,
+ "stations": "Kirby Vision,Xuesen's Inheritance,Roelofs Station,Campbell Vision"
+ },
+ {
+ "name": "HR 3954",
+ "pos_x": -36.28125,
+ "pos_y": 126.75,
+ "pos_z": -100,
+ "stations": "Hurston Stop"
+ },
+ {
+ "name": "HR 3991",
+ "pos_x": 71.8125,
+ "pos_y": 51,
+ "pos_z": -20.59375,
+ "stations": "MacLean Stop,Doi Works"
+ },
+ {
+ "name": "HR 404",
+ "pos_x": -31.34375,
+ "pos_y": -140.9375,
+ "pos_z": -48.03125,
+ "stations": "Wasden Enterprise,Bus Penal colony"
+ },
+ {
+ "name": "HR 4062",
+ "pos_x": -89.875,
+ "pos_y": 70.34375,
+ "pos_z": -67.40625,
+ "stations": "Diamond City,Bond Terminal,Marshburn Base,Okorafor Terminal,Haarsma Hub,Yurchikhin Enterprise,Baudin Colony,Spassky Survey"
+ },
+ {
+ "name": "HR 408",
+ "pos_x": 63.84375,
+ "pos_y": -226.6875,
+ "pos_z": 8.8125,
+ "stations": "Potocnik Port,Nadiradze Prospect,Sleator Terminal,Wolf Works"
+ },
+ {
+ "name": "HR 409",
+ "pos_x": -98.59375,
+ "pos_y": -43.46875,
+ "pos_z": -83.125,
+ "stations": "Howard Works,Grover's Claim,Smith Holdings"
+ },
+ {
+ "name": "HR 4130",
+ "pos_x": 125.8125,
+ "pos_y": 59.65625,
+ "pos_z": -0.71875,
+ "stations": "Garratt Ring,Favier Landing,Linge Point"
+ },
+ {
+ "name": "HR 415",
+ "pos_x": -87.875,
+ "pos_y": -61.59375,
+ "pos_z": -78.75,
+ "stations": "Franklin Landing,Saberhagen Observatory,Kier Prospect"
+ },
+ {
+ "name": "HR 4298",
+ "pos_x": 91.65625,
+ "pos_y": 43.875,
+ "pos_z": 12.3125,
+ "stations": "Foreman Station,Ampere Landing"
+ },
+ {
+ "name": "HR 4373",
+ "pos_x": 137.8125,
+ "pos_y": 63.46875,
+ "pos_z": 30.625,
+ "stations": "Haber Dock,Robinson Dock,Silverberg Refinery"
+ },
+ {
+ "name": "HR 4429",
+ "pos_x": -125.875,
+ "pos_y": 112.75,
+ "pos_z": -93.46875,
+ "stations": "Herbert Beacon,Schroeder Barracks"
+ },
+ {
+ "name": "HR 4509",
+ "pos_x": 147.84375,
+ "pos_y": -52.5,
+ "pos_z": 84.78125,
+ "stations": "Palitzsch Dock,Paola Vision,Weyn Base,Behnisch Silo"
+ },
+ {
+ "name": "HR 4547",
+ "pos_x": 96.28125,
+ "pos_y": 109.25,
+ "pos_z": 19.875,
+ "stations": "Rayhan al-Biruni Terminal,Moriarty City,Hovell Barracks,Shawl Escape"
+ },
+ {
+ "name": "HR 4548",
+ "pos_x": 83.1875,
+ "pos_y": 51.09375,
+ "pos_z": 28.28125,
+ "stations": "Acaba Station"
+ },
+ {
+ "name": "HR 4574",
+ "pos_x": 3.375,
+ "pos_y": 150.03125,
+ "pos_z": -31.875,
+ "stations": "Tokubei Station,Windt Survey,Francisco de Eliza Terminal"
+ },
+ {
+ "name": "HR 4637",
+ "pos_x": 150,
+ "pos_y": 32.15625,
+ "pos_z": 76.625,
+ "stations": "Ozanne Terminal,Asimov Orbital,Hawke Colony"
+ },
+ {
+ "name": "HR 466",
+ "pos_x": -17.15625,
+ "pos_y": -116.1875,
+ "pos_z": -42.03125,
+ "stations": "Bulychev Holdings,Blackwell Hub"
+ },
+ {
+ "name": "HR 4673",
+ "pos_x": 7.09375,
+ "pos_y": 177.8125,
+ "pos_z": -21.03125,
+ "stations": "Berezin Terminal"
+ },
+ {
+ "name": "HR 4680",
+ "pos_x": 3.40625,
+ "pos_y": 192.125,
+ "pos_z": -24.21875,
+ "stations": "Bretnor Survey,Regiomontanus Port"
+ },
+ {
+ "name": "HR 4710",
+ "pos_x": 139.3125,
+ "pos_y": -14.625,
+ "pos_z": 82.1875,
+ "stations": "Leckie Dock,Ocampo Works"
+ },
+ {
+ "name": "HR 4720",
+ "pos_x": 135.90625,
+ "pos_y": -9.0625,
+ "pos_z": 80.25,
+ "stations": "Tarelkin Port,Norgay Arsenal"
+ },
+ {
+ "name": "HR 4721",
+ "pos_x": 145.6875,
+ "pos_y": 59.8125,
+ "pos_z": 78.53125,
+ "stations": "Barnes Reach"
+ },
+ {
+ "name": "HR 4734",
+ "pos_x": 73.59375,
+ "pos_y": 20.28125,
+ "pos_z": 41.21875,
+ "stations": "Key Enterprise,Erdos Observatory,Hovell Station,Keyes City,Siegel Vision"
+ },
+ {
+ "name": "HR 4749",
+ "pos_x": 118.8125,
+ "pos_y": 14.625,
+ "pos_z": 69.03125,
+ "stations": "Abnett Enterprise,Hoyle Dock,Kessel Ring,Brunner Ring,Brillant Hub,Brosnan Terminal,Doctorow Ring,Dutton Penal colony,Scully-Power Lab"
+ },
+ {
+ "name": "HR 4776",
+ "pos_x": 73.4375,
+ "pos_y": 93.28125,
+ "pos_z": 37.375,
+ "stations": "Bond Beacon,Carter Terminal,Csoma Dock"
+ },
+ {
+ "name": "HR 4803",
+ "pos_x": 79.09375,
+ "pos_y": 64.84375,
+ "pos_z": 45.5,
+ "stations": "Ham Station,Sadi Carnot Hub,Kelly Dock,Olivas Orbital,Merbold Dock,Jones Laboratory,Afanasyev Gateway,Sheffield Penal colony,Gentle Lab"
+ },
+ {
+ "name": "HR 4926",
+ "pos_x": 13.4375,
+ "pos_y": 126.40625,
+ "pos_z": 16.09375,
+ "stations": "Yano Orbital,Velho Installation,Cayley Port,Hopkinson's Folly"
+ },
+ {
+ "name": "HR 4934",
+ "pos_x": -61.28125,
+ "pos_y": 96.96875,
+ "pos_z": -36.25,
+ "stations": "Denton Reformatory"
+ },
+ {
+ "name": "HR 494",
+ "pos_x": 35.40625,
+ "pos_y": -130.78125,
+ "pos_z": -5.875,
+ "stations": "Jordan Orbital,Leckie Port,Shalatula City,Zoline Port,Birkhoff Port,MacLeod Enterprise,Robinson Orbital,Dillon Hub,Sawyer Orbital,Martins Dock,Graham Vision,Anderson Horizons"
+ },
+ {
+ "name": "HR 4979",
+ "pos_x": 48.28125,
+ "pos_y": 28.03125,
+ "pos_z": 37.75,
+ "stations": "Iqbal Hub,Macarthur Depot"
+ },
+ {
+ "name": "HR 5000",
+ "pos_x": 102.1875,
+ "pos_y": -5.96875,
+ "pos_z": 74,
+ "stations": "Virchow Enterprise,Tuan Settlement,Frick Enterprise"
+ },
+ {
+ "name": "HR 5025",
+ "pos_x": -35.71875,
+ "pos_y": 202.34375,
+ "pos_z": 3,
+ "stations": "Harding Holdings"
+ },
+ {
+ "name": "HR 5082",
+ "pos_x": 90.21875,
+ "pos_y": -30.0625,
+ "pos_z": 64.21875,
+ "stations": "Molchanov Installation,Tank Plant,Morris Depot"
+ },
+ {
+ "name": "HR 5098",
+ "pos_x": 100.125,
+ "pos_y": 87.53125,
+ "pos_z": 97.3125,
+ "stations": "Neumann Horizons"
+ },
+ {
+ "name": "HR 5113",
+ "pos_x": 90.9375,
+ "pos_y": 0.75,
+ "pos_z": 72.8125,
+ "stations": "Freas Mine,Kibalchich Vision"
+ },
+ {
+ "name": "HR 5128",
+ "pos_x": 89.53125,
+ "pos_y": 79.53125,
+ "pos_z": 91.8125,
+ "stations": "Wescott Station,Smith Dock"
+ },
+ {
+ "name": "HR 5156",
+ "pos_x": 16.5,
+ "pos_y": 109.5625,
+ "pos_z": 42.96875,
+ "stations": "Herrington Orbital,Bose Terminal,Siemens Port,Bertin Reach,Butcher Vision,Rawat Installation,Berezin's Inheritance,Grissom Reach"
+ },
+ {
+ "name": "HR 5183",
+ "pos_x": 15.84375,
+ "pos_y": 93.46875,
+ "pos_z": 40.90625,
+ "stations": "Sullivan Port,MacVicar Base,Murray Hub,Stephenson Terminal"
+ },
+ {
+ "name": "HR 5243",
+ "pos_x": 2.09375,
+ "pos_y": 75.9375,
+ "pos_z": 28.3125,
+ "stations": "Paulo da Gama Station,Shaikh Terminal,Waldrop Point,Holdstock Platform,Spielberg's Inheritance"
+ },
+ {
+ "name": "HR 5307",
+ "pos_x": 21.96875,
+ "pos_y": 121.96875,
+ "pos_z": 75.125,
+ "stations": "Hopkins Enterprise,Halley Gateway,Hopkins Terminal,Thomas Observatory,Gibson Bastion"
+ },
+ {
+ "name": "HR 5322",
+ "pos_x": 33.1875,
+ "pos_y": 108.3125,
+ "pos_z": 81.875,
+ "stations": "Rothfuss Vision"
+ },
+ {
+ "name": "HR 5335",
+ "pos_x": -55.6875,
+ "pos_y": 137.9375,
+ "pos_z": 12.34375,
+ "stations": "Fu Enterprise,Huberath Orbital,Meaney Orbital,Houtman Landing,Cardano Vision"
+ },
+ {
+ "name": "HR 5347",
+ "pos_x": -58.15625,
+ "pos_y": 151.3125,
+ "pos_z": 17.875,
+ "stations": "McQuay Terminal,Green Port"
+ },
+ {
+ "name": "HR 5349",
+ "pos_x": 114,
+ "pos_y": -1.59375,
+ "pos_z": 108.9375,
+ "stations": "Ali Enterprise,McCulley Orbital,Gooch Orbital,Green Terminal,Aldiss Prospect"
+ },
+ {
+ "name": "HR 5436",
+ "pos_x": -63.5625,
+ "pos_y": 80.0625,
+ "pos_z": -17.3125,
+ "stations": "Low Ring,Levinson Gateway,Emshwiller City,Loncke Enterprise,Bernoulli Orbital"
+ },
+ {
+ "name": "HR 5451",
+ "pos_x": -54.3125,
+ "pos_y": 78.25,
+ "pos_z": -7.8125,
+ "stations": "Dantec City,Fraser Station,Macdonald Hub,Mieville City,Selous Orbital"
+ },
+ {
+ "name": "HR 5457",
+ "pos_x": 68.8125,
+ "pos_y": 23.59375,
+ "pos_z": 87.9375,
+ "stations": "Sinclair Colony,Reynolds Depot"
+ },
+ {
+ "name": "HR 5465",
+ "pos_x": 94.4375,
+ "pos_y": 2,
+ "pos_z": 102.21875,
+ "stations": "Meaney Arena"
+ },
+ {
+ "name": "HR 5473",
+ "pos_x": -15.0625,
+ "pos_y": 144.21875,
+ "pos_z": 80.1875,
+ "stations": "Rand Station,Porges Hub,Ing Enterprise,Schade Enterprise"
+ },
+ {
+ "name": "HR 5482",
+ "pos_x": 109.9375,
+ "pos_y": -8.84375,
+ "pos_z": 113.125,
+ "stations": "Barba Port,Papin Orbital,Watson Base,Solovyev Terminal,Cousteau Relay"
+ },
+ {
+ "name": "HR 5491",
+ "pos_x": 157.53125,
+ "pos_y": -92.90625,
+ "pos_z": 107.59375,
+ "stations": "Kagawa Colony,Maine Depot,Lee Dock"
+ },
+ {
+ "name": "HR 5492",
+ "pos_x": -81.375,
+ "pos_y": 103.375,
+ "pos_z": -16.84375,
+ "stations": "Pontes Survey,Grunsfeld Survey,Volkov Port"
+ },
+ {
+ "name": "HR 5504",
+ "pos_x": 44.96875,
+ "pos_y": 74.75,
+ "pos_z": 102.40625,
+ "stations": "Bulmer Port"
+ },
+ {
+ "name": "HR 5529",
+ "pos_x": -54.125,
+ "pos_y": 118,
+ "pos_z": 27.40625,
+ "stations": "Chadwick Terminal,Poindexter Gateway,Leslie Laboratory"
+ },
+ {
+ "name": "HR 5542",
+ "pos_x": 54,
+ "pos_y": 53.84375,
+ "pos_z": 102.34375,
+ "stations": "Lubin Landing"
+ },
+ {
+ "name": "HR 5550",
+ "pos_x": -23.96875,
+ "pos_y": 133.0625,
+ "pos_z": 76.5625,
+ "stations": "Wohler Gateway,Ejeta Survey,Shaw Port,Gibson Hub,Veblen Base"
+ },
+ {
+ "name": "HR 5630",
+ "pos_x": -42.21875,
+ "pos_y": 83.9375,
+ "pos_z": 25.28125,
+ "stations": "Beaufoy Orbital,Barentsz Depot"
+ },
+ {
+ "name": "HR 5632",
+ "pos_x": 51.5625,
+ "pos_y": -4.59375,
+ "pos_z": 59.5625,
+ "stations": "Roentgen Orbital,Leclerc Ring,Liska Station"
+ },
+ {
+ "name": "HR 5663",
+ "pos_x": 88.34375,
+ "pos_y": 21.625,
+ "pos_z": 134.84375,
+ "stations": "Stevin Enterprise,Grijalva Vision,Luiken Point,King Lab"
+ },
+ {
+ "name": "HR 5706",
+ "pos_x": 0.09375,
+ "pos_y": 77.75,
+ "pos_z": 83.78125,
+ "stations": "Wul Orbital,Ing Orbital,Maybury Arsenal"
+ },
+ {
+ "name": "HR 571",
+ "pos_x": 84.53125,
+ "pos_y": -126.28125,
+ "pos_z": 29.09375,
+ "stations": "Kopal Station,Pilyugin Vision,Bainbridge Market,Schmidt Dock,Shavyrin Terminal,Garrido Vision"
+ },
+ {
+ "name": "HR 5716",
+ "pos_x": -95.71875,
+ "pos_y": 144.9375,
+ "pos_z": 30.09375,
+ "stations": "Andrews Gateway,Pike Terminal"
+ },
+ {
+ "name": "HR 5740",
+ "pos_x": -39.1875,
+ "pos_y": 107.875,
+ "pos_z": 70.1875,
+ "stations": "Morukov Hub,Lyell Hub,Atiyah Lab"
+ },
+ {
+ "name": "HR 5751",
+ "pos_x": 72.65625,
+ "pos_y": 41.65625,
+ "pos_z": 153.625,
+ "stations": "Willis Oasis,Lander Survey"
+ },
+ {
+ "name": "HR 5758",
+ "pos_x": -28.15625,
+ "pos_y": 121.59375,
+ "pos_z": 108.125,
+ "stations": "Fidalgo Prospect,Hughes Terminal,Samuda Enterprise"
+ },
+ {
+ "name": "HR 5791",
+ "pos_x": -17.25,
+ "pos_y": 124.6875,
+ "pos_z": 134.125,
+ "stations": "Robinson Dock,Samuda Enterprise,Cole Hub"
+ },
+ {
+ "name": "HR 5813",
+ "pos_x": -52.6875,
+ "pos_y": 96.15625,
+ "pos_z": 48.625,
+ "stations": "Lyakhov Camp,Dashiell Hub,Neumann Dock,Burgess Laboratory"
+ },
+ {
+ "name": "HR 5816",
+ "pos_x": 2.59375,
+ "pos_y": 46.84375,
+ "pos_z": 66.1875,
+ "stations": "Foreman Survey,Barentsz Market,Adams Gateway"
+ },
+ {
+ "name": "HR 5930",
+ "pos_x": 10.1875,
+ "pos_y": 78.09375,
+ "pos_z": 146.84375,
+ "stations": "Crown Orbital,Foreman Enterprise,Wiener Hub"
+ },
+ {
+ "name": "HR 5960",
+ "pos_x": -75.28125,
+ "pos_y": 79.28125,
+ "pos_z": 6.40625,
+ "stations": "Ali Orbital,Chiang's Inheritance,Payette Dock,Laing Survey"
+ },
+ {
+ "name": "HR 5970",
+ "pos_x": 47.5625,
+ "pos_y": 45.65625,
+ "pos_z": 167.5625,
+ "stations": "Russ Base,Cooper Dock"
+ },
+ {
+ "name": "HR 5975",
+ "pos_x": 41.15625,
+ "pos_y": 22,
+ "pos_z": 114.375,
+ "stations": "Boole Gateway,Selous Gateway,McKay Barracks"
+ },
+ {
+ "name": "HR 5991",
+ "pos_x": 56.09375,
+ "pos_y": 33.21875,
+ "pos_z": 166.375,
+ "stations": "Fozard Terminal"
+ },
+ {
+ "name": "HR 5996",
+ "pos_x": 1.96875,
+ "pos_y": 39.53125,
+ "pos_z": 79.03125,
+ "stations": "Bailey Orbital,Powers Stop,Tenn Barracks"
+ },
+ {
+ "name": "HR 6067",
+ "pos_x": -20.21875,
+ "pos_y": 74.40625,
+ "pos_z": 122.59375,
+ "stations": "Evangelisti Freeport"
+ },
+ {
+ "name": "HR 6077",
+ "pos_x": 33.34375,
+ "pos_y": 35.53125,
+ "pos_z": 149.65625,
+ "stations": "Ricardo Depot"
+ },
+ {
+ "name": "HR 6091",
+ "pos_x": -54.15625,
+ "pos_y": 60.875,
+ "pos_z": 27.625,
+ "stations": "Pogue Plant,Hadfield Plant,Gooch Colony,Watts Holdings"
+ },
+ {
+ "name": "HR 6177",
+ "pos_x": 53.21875,
+ "pos_y": -17.1875,
+ "pos_z": 85.5,
+ "stations": "Baudry Ring,Halley Bastion,Bradley Arena"
+ },
+ {
+ "name": "HR 6189",
+ "pos_x": -16.5625,
+ "pos_y": 51.1875,
+ "pos_z": 119.0625,
+ "stations": "McMonagle Orbital,Makarov Ring,Conrad Orbital,Hutton Terminal,Hirase Hub,Drake Terminal,Barry Arsenal,Rochon Plant,Heisenberg Port,Yurchikhin Lab"
+ },
+ {
+ "name": "HR 6201",
+ "pos_x": -39.0625,
+ "pos_y": 73.625,
+ "pos_z": 135.96875,
+ "stations": "Noon Settlement,Beliaev Penal colony"
+ },
+ {
+ "name": "HR 6202",
+ "pos_x": 1.28125,
+ "pos_y": 38.75,
+ "pos_z": 131.53125,
+ "stations": "Beckman Hub,Dutton Hub,Deere Installation"
+ },
+ {
+ "name": "HR 6269",
+ "pos_x": 26.25,
+ "pos_y": 80.03125,
+ "pos_z": 64.53125,
+ "stations": "Kimberlin Vision,Karlsefni Keep,Ellison Hub,Cabral Orbital"
+ },
+ {
+ "name": "HR 6284",
+ "pos_x": -9.5,
+ "pos_y": 36.875,
+ "pos_z": 131.625,
+ "stations": "Walker Port,Hornblower Orbital"
+ },
+ {
+ "name": "HR 6297",
+ "pos_x": 72.40625,
+ "pos_y": -23.65625,
+ "pos_z": 150.84375,
+ "stations": "Ibold's Inheritance,Nachtigal Settlement,Temple Landing"
+ },
+ {
+ "name": "HR 6301",
+ "pos_x": -93.90625,
+ "pos_y": 102.3125,
+ "pos_z": 143.03125,
+ "stations": "Kent Port,Zamyatin Point"
+ },
+ {
+ "name": "HR 6328",
+ "pos_x": -85.65625,
+ "pos_y": 78.9375,
+ "pos_z": 75.6875,
+ "stations": "Perry Dock,Soukup Port"
+ },
+ {
+ "name": "HR 6351",
+ "pos_x": -122.9375,
+ "pos_y": 104.9375,
+ "pos_z": 77.59375,
+ "stations": "Turtledove Orbital,Chern Base,Mohun Refinery"
+ },
+ {
+ "name": "HR 638",
+ "pos_x": -5.78125,
+ "pos_y": -139.53125,
+ "pos_z": -67.875,
+ "stations": "Hafner Settlement"
+ },
+ {
+ "name": "HR 6398",
+ "pos_x": 19.25,
+ "pos_y": -1.21875,
+ "pos_z": 97.875,
+ "stations": "Padalka Hub,Czerny Landing"
+ },
+ {
+ "name": "HR 6421",
+ "pos_x": -114.78125,
+ "pos_y": 80.71875,
+ "pos_z": -4.875,
+ "stations": "Julian Legacy,Serebrov Terminal,Bayliss Point"
+ },
+ {
+ "name": "HR 6467",
+ "pos_x": -102.78125,
+ "pos_y": 73.15625,
+ "pos_z": 28.5,
+ "stations": "Alenquer Vision,McKee Works,White Camp"
+ },
+ {
+ "name": "HR 6489",
+ "pos_x": -70.78125,
+ "pos_y": 60.75,
+ "pos_z": 178.8125,
+ "stations": "Goonan Arena,Clair Keep"
+ },
+ {
+ "name": "HR 6496",
+ "pos_x": -29.0625,
+ "pos_y": 28.875,
+ "pos_z": 135.8125,
+ "stations": "Ivanishin Ring,Bakewell Station"
+ },
+ {
+ "name": "HR 6507",
+ "pos_x": -74.625,
+ "pos_y": 59.6875,
+ "pos_z": 169.375,
+ "stations": "Fu Landing,Nicholson Colony,Narvaez Survey,Szameit Survey"
+ },
+ {
+ "name": "HR 6534",
+ "pos_x": -49.28125,
+ "pos_y": 37.59375,
+ "pos_z": 144.09375,
+ "stations": "Marley Station,Cooper Enterprise,Malchiodi Landing,Nicholson Keep"
+ },
+ {
+ "name": "HR 6541",
+ "pos_x": -75.40625,
+ "pos_y": 51.96875,
+ "pos_z": 81.8125,
+ "stations": "Ashton Orbital,Vance Port,Waldeck City,Fourier Terminal,Benyovszky City,Cogswell City,Nielsen Hub,Rolland Settlement"
+ },
+ {
+ "name": "HR 6552",
+ "pos_x": 155.15625,
+ "pos_y": -97.71875,
+ "pos_z": 122.21875,
+ "stations": "Wagner Relay,Allen Enterprise"
+ },
+ {
+ "name": "HR 6565",
+ "pos_x": 82.40625,
+ "pos_y": -51.375,
+ "pos_z": 103,
+ "stations": "Naubakht Station,Bounds Survey,Moresby Holdings,Jones Hub,Engle City"
+ },
+ {
+ "name": "HR 6594",
+ "pos_x": -68.125,
+ "pos_y": 42.34375,
+ "pos_z": 80.5625,
+ "stations": "Haberlandt Penal colony,Kennicott Platform,Lem Landing"
+ },
+ {
+ "name": "HR 6598",
+ "pos_x": -124.6875,
+ "pos_y": 78.5625,
+ "pos_z": -22.03125,
+ "stations": "Flynn Colony,Bailey's Claim,McCarthy Settlement"
+ },
+ {
+ "name": "HR 6649",
+ "pos_x": 18.03125,
+ "pos_y": -15.28125,
+ "pos_z": 100.34375,
+ "stations": "Gibson Station,Willis Relay"
+ },
+ {
+ "name": "HR 6669",
+ "pos_x": -88.71875,
+ "pos_y": 51.03125,
+ "pos_z": 39.21875,
+ "stations": "Parker Hub,Deb Mines,Pontes Arsenal"
+ },
+ {
+ "name": "HR 6680",
+ "pos_x": -6.84375,
+ "pos_y": -6.59375,
+ "pos_z": 167.0625,
+ "stations": "McAllaster Port"
+ },
+ {
+ "name": "HR 6767",
+ "pos_x": -157.21875,
+ "pos_y": 81.0625,
+ "pos_z": 60.71875,
+ "stations": "Dickson Dock,Cook Terminal,Williams Station,Koontz's Pride,Francisco de Almeida Holdings,Payne Landing"
+ },
+ {
+ "name": "HR 6797",
+ "pos_x": -78.21875,
+ "pos_y": 26.84375,
+ "pos_z": 128.78125,
+ "stations": "Jordan Settlement,Plante Settlement"
+ },
+ {
+ "name": "HR 6828",
+ "pos_x": 34,
+ "pos_y": -27.34375,
+ "pos_z": 61.1875,
+ "stations": "Celsius Hub,Everest Relay,Leclerc Observatory"
+ },
+ {
+ "name": "HR 6831",
+ "pos_x": -108.09375,
+ "pos_y": 46.90625,
+ "pos_z": 71.8125,
+ "stations": "Pirsan Terminal,Griffiths Depot,Novitski Terminal"
+ },
+ {
+ "name": "HR 6836",
+ "pos_x": -7.53125,
+ "pos_y": -11.03125,
+ "pos_z": 98.53125,
+ "stations": "Popovich Terminal,Culbertson Dock,Bella Dock,Townshend City,Hubble Dock,Tolstoi Silo"
+ },
+ {
+ "name": "HR 687",
+ "pos_x": -91.3125,
+ "pos_y": -47.03125,
+ "pos_z": -113.90625,
+ "stations": "Palmer Refinery,Farrer Point,Sinclair Relay"
+ },
+ {
+ "name": "HR 6907",
+ "pos_x": -8.3125,
+ "pos_y": -18.84375,
+ "pos_z": 117.59375,
+ "stations": "Melnick City,Kelleam Prospect,Durrance Orbital"
+ },
+ {
+ "name": "HR 692",
+ "pos_x": 0.1875,
+ "pos_y": -85.84375,
+ "pos_z": -44.625,
+ "stations": "Zindell Gateway,Pond Horizons,Morgan Installation,Burke's Claim,Garfinkle Terminal"
+ },
+ {
+ "name": "HR 6948",
+ "pos_x": 9.78125,
+ "pos_y": -29.875,
+ "pos_z": 116.4375,
+ "stations": "Atwater Terminal,Born Terminal,Johnson Station,Cowper Base"
+ },
+ {
+ "name": "HR 6950",
+ "pos_x": -83.84375,
+ "pos_y": 25.90625,
+ "pos_z": 70.84375,
+ "stations": "Stith Dock"
+ },
+ {
+ "name": "HR 6987",
+ "pos_x": -65.40625,
+ "pos_y": 10.875,
+ "pos_z": 85.125,
+ "stations": "Taylor City,Creighton Port,Alexandrov Arsenal"
+ },
+ {
+ "name": "HR 6999",
+ "pos_x": -75.71875,
+ "pos_y": 2.375,
+ "pos_z": 136.875,
+ "stations": "Pontes Station,Slayton Port,Musa al-Khwarizmi Survey,Barjavel Relay"
+ },
+ {
+ "name": "HR 7012",
+ "pos_x": 41.46875,
+ "pos_y": -38.25,
+ "pos_z": 74.0625,
+ "stations": "Pratchett Plant,Lee Prospect,Heck Prospect,Cook Enterprise"
+ },
+ {
+ "name": "HR 7047",
+ "pos_x": -113.3125,
+ "pos_y": 28.59375,
+ "pos_z": 83.03125,
+ "stations": "Grant Dock,Kafka Terminal,Doi Base,Soper Penal colony,The Den"
+ },
+ {
+ "name": "HR 706",
+ "pos_x": 39.3125,
+ "pos_y": -93.09375,
+ "pos_z": -11.625,
+ "stations": "Chernykh Port,Wright Terminal,Rubin Dock,Riccioli Terminal,Laming Orbital,Glushko Vision,Laurent Station,Valz Orbital,Kopal Orbital"
+ },
+ {
+ "name": "HR 7079",
+ "pos_x": -74.59375,
+ "pos_y": 17.4375,
+ "pos_z": 54.09375,
+ "stations": "Ochoa Terminal"
+ },
+ {
+ "name": "HR 7169",
+ "pos_x": -0.21875,
+ "pos_y": -43.875,
+ "pos_z": 132.34375,
+ "stations": "Wrangel Port,Crichton Prospect,Euthymenes Orbital,Giles Station"
+ },
+ {
+ "name": "HR 7221",
+ "pos_x": 58.53125,
+ "pos_y": -55.8125,
+ "pos_z": 91.25,
+ "stations": "Jewitt Port,Veron City"
+ },
+ {
+ "name": "HR 7263",
+ "pos_x": -113.84375,
+ "pos_y": 14.28125,
+ "pos_z": 81.34375,
+ "stations": "Ericsson Settlement,Deb Dock"
+ },
+ {
+ "name": "HR 7291",
+ "pos_x": -20.625,
+ "pos_y": -25.375,
+ "pos_z": 83.6875,
+ "stations": "Lander Port,Vance Orbital,Yourkevitch Hub,Hale Vision,Hadfield Installation"
+ },
+ {
+ "name": "HR 7294",
+ "pos_x": -75.25,
+ "pos_y": 23.4375,
+ "pos_z": 12.125,
+ "stations": "Springer Market,Gotlieb Beacon,Utley Barracks"
+ },
+ {
+ "name": "HR 7297",
+ "pos_x": 36.1875,
+ "pos_y": -64.1875,
+ "pos_z": 127.1875,
+ "stations": "Reeves Platform,Albitzky Prospect,Stein Point"
+ },
+ {
+ "name": "HR 7327",
+ "pos_x": -47.71875,
+ "pos_y": -52.09375,
+ "pos_z": 166.21875,
+ "stations": "Friend Enterprise,Gabriel Station,Dyr's Folly,Hiraga Gateway"
+ },
+ {
+ "name": "HR 7386",
+ "pos_x": -76.5625,
+ "pos_y": 5.90625,
+ "pos_z": 45.65625,
+ "stations": "Bulleid Orbital"
+ },
+ {
+ "name": "HR 7451",
+ "pos_x": -78.84375,
+ "pos_y": 20.4375,
+ "pos_z": 8.75,
+ "stations": "Gillekens Station,Goddard Terminal,Comino Terminal,Brady Port,Hire Port,Cheli Gateway"
+ },
+ {
+ "name": "HR 7468",
+ "pos_x": -127.75,
+ "pos_y": 25.75,
+ "pos_z": 27.5,
+ "stations": "Yu Holdings,Williams Reach,Rand Penal colony"
+ },
+ {
+ "name": "HR 7484",
+ "pos_x": -128.375,
+ "pos_y": 35.625,
+ "pos_z": 5.75,
+ "stations": "Dzhanibekov Gateway,Malocello Base,Forstchen Installation"
+ },
+ {
+ "name": "HR 755",
+ "pos_x": 97.25,
+ "pos_y": -162.6875,
+ "pos_z": 0.40625,
+ "stations": "McQuarrie Vision,Courvoisier Dock,Seamans Dock,Blaschke Installation,Mayer Keep"
+ },
+ {
+ "name": "HR 7579",
+ "pos_x": 107.46875,
+ "pos_y": -120.03125,
+ "pos_z": 162.34375,
+ "stations": "Bisnovatyi-Kogan Depot"
+ },
+ {
+ "name": "HR 7605",
+ "pos_x": -6.625,
+ "pos_y": -80.125,
+ "pos_z": 142.34375,
+ "stations": "Islam Prospect,Cheranovsky Dock"
+ },
+ {
+ "name": "HR 7674",
+ "pos_x": 18.4375,
+ "pos_y": -41.90625,
+ "pos_z": 61.1875,
+ "stations": "Barbaro Plant,Wnuk-Lipinski Terminal,Mitra City,Tenn Terminal"
+ },
+ {
+ "name": "HR 7698",
+ "pos_x": 163.75,
+ "pos_y": -123.25,
+ "pos_z": 137.65625,
+ "stations": "Hulse Terminal,Clark Station"
+ },
+ {
+ "name": "HR 7729",
+ "pos_x": -14.78125,
+ "pos_y": -78,
+ "pos_z": 122.125,
+ "stations": "Bushkov Orbital,Krusvar Vision,Back Orbital"
+ },
+ {
+ "name": "HR 7749",
+ "pos_x": 11.0625,
+ "pos_y": -57.1875,
+ "pos_z": 81.71875,
+ "stations": "Pythagoras Hub,Smith Terminal,Gagnan Enterprise,Andreas' Progress,Maddox Terminal"
+ },
+ {
+ "name": "HR 7756",
+ "pos_x": -110.1875,
+ "pos_y": 10.96875,
+ "pos_z": 15.0625,
+ "stations": "Bulmer Enterprise,Watts Colony"
+ },
+ {
+ "name": "HR 7766",
+ "pos_x": 15.25,
+ "pos_y": -58.40625,
+ "pos_z": 81.1875,
+ "stations": "Jordan Arsenal,Tarski Gateway,Macgregor Enterprise,Markov Hub,Buckell Installation,Clair City,Sohl Station,Crook Ring,Gregory Beacon"
+ },
+ {
+ "name": "HR 7793",
+ "pos_x": -70.1875,
+ "pos_y": -19.40625,
+ "pos_z": 45.0625,
+ "stations": "Benyovszky Ring,Khan Barracks"
+ },
+ {
+ "name": "HR 780",
+ "pos_x": 69.46875,
+ "pos_y": -163.125,
+ "pos_z": -32.75,
+ "stations": "Denning Terminal,Orban Vision,Nemere Laboratory,Madsen Keep"
+ },
+ {
+ "name": "HR 7808",
+ "pos_x": -8.34375,
+ "pos_y": -69.625,
+ "pos_z": 99.53125,
+ "stations": "Hatanaka Prospect,Haack Hub,Hafner Port,Morrow Holdings,Lowry Enterprise"
+ },
+ {
+ "name": "HR 783",
+ "pos_x": -21.84375,
+ "pos_y": -96.25,
+ "pos_z": -86.9375,
+ "stations": "Lyulka City,Roth Hub,Rich Orbital,Stiles Ring"
+ },
+ {
+ "name": "HR 784",
+ "pos_x": 2.53125,
+ "pos_y": -60.34375,
+ "pos_z": -37.28125,
+ "stations": "Rominger Station,Schrodinger Dock,Jahn Station,Cameron Enterprise,Morey Port,Haipeng Town,Nowak Colony,Blenkinsop Port,Foale Port,Galvani Depot,Hennen Enterprise,Ohm Colony"
+ },
+ {
+ "name": "HR 7907",
+ "pos_x": -86.84375,
+ "pos_y": -34.46875,
+ "pos_z": 56.78125,
+ "stations": "Ham Hub"
+ },
+ {
+ "name": "HR 7925",
+ "pos_x": -104.53125,
+ "pos_y": 21.0625,
+ "pos_z": -12.1875,
+ "stations": "Bethke Escape,Hausdorff Port,Williamson Port,Levy Holdings,Williams Base,Steinmuller Mine,Utley Settlement"
+ },
+ {
+ "name": "HR 8019",
+ "pos_x": 115.40625,
+ "pos_y": -158,
+ "pos_z": 171.375,
+ "stations": "Halley Colony,Lyne Terminal,Arkhangelsky Survey"
+ },
+ {
+ "name": "HR 8031",
+ "pos_x": -13.09375,
+ "pos_y": -86.5625,
+ "pos_z": 97.84375,
+ "stations": "Womack Dock,Rondon Horizons,Scithers Terminal,Cartier Depot"
+ },
+ {
+ "name": "HR 8061",
+ "pos_x": 33.46875,
+ "pos_y": -37.9375,
+ "pos_z": 39.9375,
+ "stations": "Ehrlich Terminal,Bowen Dock,Libby Hub,Bridges Hub,Hugh Prospect,Harvey Hub,Frimout Gateway"
+ },
+ {
+ "name": "HR 8104",
+ "pos_x": -4.625,
+ "pos_y": -74.78125,
+ "pos_z": 77.09375,
+ "stations": "Anvil Arsenal,Vetulani Port,Bulleid Ring,Diesel Ring,Buchli Enterprise,Taylor Orbital,Hedley Orbital,Asprin Mine,Malpighi Terminal,Tsunenaga Vision,Krikalev Port,Gemar Orbital,Yamazaki Base,Burkin Vision"
+ },
+ {
+ "name": "HR 8133",
+ "pos_x": -133.875,
+ "pos_y": 26.28125,
+ "pos_z": -28.96875,
+ "stations": "Leeuwenhoek Gateway,Meikle Dock,Burbank Gateway,Smeaton Dock,Payton Gateway,Tryggvason Port,Ricardo Gateway,Amundsen Lab,Ostwald Relay,Bondar Camp"
+ },
+ {
+ "name": "HR 816",
+ "pos_x": -21.375,
+ "pos_y": -117.78125,
+ "pos_z": -106.375,
+ "stations": "Laumer Platform,Piserchia Landing,Belyanin Plant"
+ },
+ {
+ "name": "HR 8170",
+ "pos_x": -87.25,
+ "pos_y": -10.46875,
+ "pos_z": 6.40625,
+ "stations": "Fiennes Vision,Vasilyev Vision,Alexander Port,Cremona Point,Bell Installation"
+ },
+ {
+ "name": "HR 8202",
+ "pos_x": 2.375,
+ "pos_y": -133.875,
+ "pos_z": 126.28125,
+ "stations": "Behnisch Terminal,Zaschka Hub,Heiles Orbital,Thierree Survey"
+ },
+ {
+ "name": "HR 8208",
+ "pos_x": -109.03125,
+ "pos_y": -5.34375,
+ "pos_z": -1.53125,
+ "stations": "Bova Orbital,Sturgeon Station,King Dock,Krusvar Base,Leeuwenhoek Survey,Simpson Landing,Elder Landing"
+ },
+ {
+ "name": "HR 8210",
+ "pos_x": -132.34375,
+ "pos_y": -57.25,
+ "pos_z": 45.5625,
+ "stations": "Hyecho Port"
+ },
+ {
+ "name": "HR 8212",
+ "pos_x": -58.8125,
+ "pos_y": -75.96875,
+ "pos_z": 66.78125,
+ "stations": "Ostrander Relay,Petaja Landing"
+ },
+ {
+ "name": "HR 8250",
+ "pos_x": -185.71875,
+ "pos_y": -74.96875,
+ "pos_z": 49.5625,
+ "stations": "Thurston Beacon,Ford Mine"
+ },
+ {
+ "name": "HR 826",
+ "pos_x": 33.03125,
+ "pos_y": -139.1875,
+ "pos_z": -63.875,
+ "stations": "Bradfield Ring,Franke Installation,Schulhof City"
+ },
+ {
+ "name": "HR 8268",
+ "pos_x": -20.28125,
+ "pos_y": -110.6875,
+ "pos_z": 94.0625,
+ "stations": "Linenger Terminal,Willis Hub,Bose Terminal,Cleve Market,Lind Terminal"
+ },
+ {
+ "name": "HR 827",
+ "pos_x": 28.75,
+ "pos_y": -114.96875,
+ "pos_z": -51.53125,
+ "stations": "Smith Station,Asire Ring,Windt Vision,Naddoddur Hub,Cartan Horizons,Bridger Terminal,Hedin City,Cousin Hub,Dayuan Terminal,Arkhangelsky Vision"
+ },
+ {
+ "name": "HR 8276",
+ "pos_x": -114,
+ "pos_y": -52.3125,
+ "pos_z": 32.875,
+ "stations": "Britnev Dock,Chebyshev Relay,Rasmussen Dock"
+ },
+ {
+ "name": "HR 8352",
+ "pos_x": 52.75,
+ "pos_y": -107.5625,
+ "pos_z": 92.8125,
+ "stations": "Oshima Platform,Nansen Lab,Ziewe Landing"
+ },
+ {
+ "name": "HR 8380",
+ "pos_x": 115.6875,
+ "pos_y": -123.78125,
+ "pos_z": 113.8125,
+ "stations": "Newcomb Terminal,Shinn Plant,Jones Landing"
+ },
+ {
+ "name": "HR 8423",
+ "pos_x": -105.375,
+ "pos_y": 48.25,
+ "pos_z": -55.53125,
+ "stations": "Thuot City,Singer Port,Haldeman Installation"
+ },
+ {
+ "name": "HR 8444",
+ "pos_x": -24.4375,
+ "pos_y": -170.15625,
+ "pos_z": 114.8125,
+ "stations": "Maskelyne Vision,Abnett Point,Collins Market"
+ },
+ {
+ "name": "HR 8461",
+ "pos_x": -147.75,
+ "pos_y": -95.59375,
+ "pos_z": 34.09375,
+ "stations": "Davy Survey"
+ },
+ {
+ "name": "HR 8472",
+ "pos_x": -118.84375,
+ "pos_y": 1.125,
+ "pos_z": -26.875,
+ "stations": "Pryor Gateway,Neville Ring,Gregory Gateway,Kennicott Installation,Walker Camp,Fairbairn's Progress"
+ },
+ {
+ "name": "HR 8474",
+ "pos_x": -97.96875,
+ "pos_y": 21.3125,
+ "pos_z": -36.125,
+ "stations": "Hillary Silo,Haisheng Orbital,Carleson Refinery,Shukor Gateway,Bunsen Gateway,Artyukhin Landing"
+ },
+ {
+ "name": "HR 8514",
+ "pos_x": -74.21875,
+ "pos_y": -64.1875,
+ "pos_z": 23.46875,
+ "stations": "Clarke Arsenal,Coleman Hub,Hennen Station,Bowersox Station"
+ },
+ {
+ "name": "HR 8526",
+ "pos_x": 41.78125,
+ "pos_y": -47.4375,
+ "pos_z": 40.6875,
+ "stations": "Volk Hub,Matteucci Hub,Budarin City,Hoshi Point,Reilly Station,Ochoa Enterprise,Grechko City,Romanenko Enterprise,Mastracchio City,Jemison Orbital,Salam Keep,Sladek Vista,Lockhart's Inheritance,Coelho Holdings"
+ },
+ {
+ "name": "HR 8527",
+ "pos_x": 79,
+ "pos_y": -108.78125,
+ "pos_z": 89.125,
+ "stations": "Seki Refinery,Graham Holdings,Brashear City,Napier Holdings,Tennyson d'Eyncourt Base"
+ },
+ {
+ "name": "HR 8563",
+ "pos_x": -28.5625,
+ "pos_y": -111.6875,
+ "pos_z": 60.25,
+ "stations": "Keyes' Folly,Kurtz Colony,Chaffee Landing,Poyser Installation"
+ },
+ {
+ "name": "HR 8593",
+ "pos_x": 65.625,
+ "pos_y": -171.5,
+ "pos_z": 118.65625,
+ "stations": "Houten-Groeneveld Survey,O'Neill Platform,Campbell Vision,Gorgani Installation"
+ },
+ {
+ "name": "HR 8631",
+ "pos_x": -86.375,
+ "pos_y": -67.5625,
+ "pos_z": 11.375,
+ "stations": "Nicollet Market,Lenthall Bastion,Aikin Settlement,Rochon Enterprise"
+ },
+ {
+ "name": "HR 8662",
+ "pos_x": 32.71875,
+ "pos_y": -219.375,
+ "pos_z": 127.0625,
+ "stations": "Lopez-Garcia Dock,Horrocks Dock,Bottego Arsenal"
+ },
+ {
+ "name": "HR 8666",
+ "pos_x": -177.6875,
+ "pos_y": -41.0625,
+ "pos_z": -34.84375,
+ "stations": "Nesvadba Vision,Sarmiento de Gamboa Bastion"
+ },
+ {
+ "name": "HR 8671",
+ "pos_x": -3.78125,
+ "pos_y": -196.65625,
+ "pos_z": 102.34375,
+ "stations": "Barnwell Orbital,Whitcomb Port,Helin Vision,Millosevich Refinery"
+ },
+ {
+ "name": "HR 8718",
+ "pos_x": -117.96875,
+ "pos_y": -43.9375,
+ "pos_z": -18.875,
+ "stations": "Ramon Dock,Kidman Dock,Turtledove Refinery"
+ },
+ {
+ "name": "HR 8737",
+ "pos_x": -75.15625,
+ "pos_y": -74.53125,
+ "pos_z": 9.09375,
+ "stations": "Savery Dock,Lamarr Port,Melnick Ring,Melvill Hub,Virchow Port,Gidzenko Orbital,Marshburn Ring,Blackman Base,Duckworth Orbital,Perrin Dock,Cheli Terminal"
+ },
+ {
+ "name": "HR 8769",
+ "pos_x": 84.125,
+ "pos_y": -125.0625,
+ "pos_z": 87.59375,
+ "stations": "Gustav Sporer Platform"
+ },
+ {
+ "name": "HR 8786",
+ "pos_x": 150,
+ "pos_y": -143.625,
+ "pos_z": 119.59375,
+ "stations": "Andoyer Landing"
+ },
+ {
+ "name": "HR 8788",
+ "pos_x": -112.25,
+ "pos_y": -87.03125,
+ "pos_z": -3.3125,
+ "stations": "Lazutkin Terminal,Shiner Survey,Boltzmann Refinery"
+ },
+ {
+ "name": "HR 8792",
+ "pos_x": -73.15625,
+ "pos_y": -54.34375,
+ "pos_z": -3.3125,
+ "stations": "Karlsefni Dock,White City,Plucker Terminal,Lethem City,Perez Enterprise"
+ },
+ {
+ "name": "HR 8793",
+ "pos_x": 26.84375,
+ "pos_y": -112.0625,
+ "pos_z": 59.5,
+ "stations": "Banneker Dock"
+ },
+ {
+ "name": "HR 8799",
+ "pos_x": -104.3125,
+ "pos_y": -74.84375,
+ "pos_z": -6.46875,
+ "stations": "Pailes Vision,Duckworth Station"
+ },
+ {
+ "name": "HR 8809",
+ "pos_x": 94.875,
+ "pos_y": -153.59375,
+ "pos_z": 102.25,
+ "stations": "Adams Gateway,Tomita Base,Johnson Orbital,Korolev Dock,Langley Depot"
+ },
+ {
+ "name": "HR 8829",
+ "pos_x": 38.1875,
+ "pos_y": -74.84375,
+ "pos_z": 45.875,
+ "stations": "Ashbrook Dock,North Installation,Elbakyan Survey"
+ },
+ {
+ "name": "HR 8845",
+ "pos_x": -120.3125,
+ "pos_y": -79.5,
+ "pos_z": -16.125,
+ "stations": "Dedman Orbital,Sharp Vision,Tevis Gateway"
+ },
+ {
+ "name": "HR 8970",
+ "pos_x": -114.96875,
+ "pos_y": -134.59375,
+ "pos_z": -12.75,
+ "stations": "Shaara Arsenal,Vries Holdings"
+ },
+ {
+ "name": "HR 8977",
+ "pos_x": -123.8125,
+ "pos_y": -57.71875,
+ "pos_z": -40.4375,
+ "stations": "Mendeleev Settlement,McDivitt Depot,Stanley Relay"
+ },
+ {
+ "name": "HR 8993",
+ "pos_x": 35.65625,
+ "pos_y": -209.40625,
+ "pos_z": 76.3125,
+ "stations": "Bolton Mines,Bailey Relay,Allen Colony,Henry Terminal"
+ },
+ {
+ "name": "HR 9001",
+ "pos_x": 18.40625,
+ "pos_y": -209.03125,
+ "pos_z": 66.34375,
+ "stations": "Fox Survey,Kagawa Settlement,Huss Depot"
+ },
+ {
+ "name": "HR 9046",
+ "pos_x": 8.8125,
+ "pos_y": -86.375,
+ "pos_z": 25.03125,
+ "stations": "Savitskaya Gateway,Gotlieb Hub,Wafer's Progress"
+ },
+ {
+ "name": "HR 9060",
+ "pos_x": 94.09375,
+ "pos_y": -173.0625,
+ "pos_z": 87.1875,
+ "stations": "Celsius Vision,Arkhangelsky Station,Lagrange Vision,Stairs Market,Rustah Keep"
+ },
+ {
+ "name": "HR 9095",
+ "pos_x": -40.34375,
+ "pos_y": -194.53125,
+ "pos_z": 18.375,
+ "stations": "Common Station,Comper Orbital,Hamuy Gateway"
+ },
+ {
+ "name": "HR 913",
+ "pos_x": 6.6875,
+ "pos_y": -87.3125,
+ "pos_z": -67.5625,
+ "stations": "Thomas Holdings,Shatner Arena,Goodman Installation"
+ },
+ {
+ "name": "HR 916",
+ "pos_x": -55.5,
+ "pos_y": -64.96875,
+ "pos_z": -123.25,
+ "stations": "Ramon Relay,Locke Works"
+ },
+ {
+ "name": "HR 943",
+ "pos_x": 60.8125,
+ "pos_y": -153.34375,
+ "pos_z": -67.53125,
+ "stations": "Hildebrandt Terminal,Lewis Bastion"
+ },
+ {
+ "name": "HR 975",
+ "pos_x": -69.8125,
+ "pos_y": -62.25,
+ "pos_z": -152.3125,
+ "stations": "Varley Installation"
+ },
+ {
+ "name": "Hraean",
+ "pos_x": 42.875,
+ "pos_y": -64.46875,
+ "pos_z": -167.65625,
+ "stations": "Bellamy Escape"
+ },
+ {
+ "name": "Hraeango",
+ "pos_x": -173.75,
+ "pos_y": -9.96875,
+ "pos_z": -42.53125,
+ "stations": "Baker Asylum"
+ },
+ {
+ "name": "Hrana",
+ "pos_x": -46.9375,
+ "pos_y": -12.375,
+ "pos_z": 94.5,
+ "stations": "Meitner City,Fisher Enterprise,Ivanchenkov Vision"
+ },
+ {
+ "name": "Hranbi",
+ "pos_x": 116.3125,
+ "pos_y": -99.75,
+ "pos_z": 17.78125,
+ "stations": "Kohoutek Installation,Dubyago Settlement,Jean Arsenal"
+ },
+ {
+ "name": "Hranit",
+ "pos_x": -11.59375,
+ "pos_y": -80.625,
+ "pos_z": -66.21875,
+ "stations": "Al-Jazari Orbital,Zhigang City,McMonagle Dock,Young Enterprise"
+ },
+ {
+ "name": "Hrans",
+ "pos_x": 115.71875,
+ "pos_y": -59.125,
+ "pos_z": -34.125,
+ "stations": "Lyulka Hangar"
+ },
+ {
+ "name": "Hranyi",
+ "pos_x": 143.59375,
+ "pos_y": 50.40625,
+ "pos_z": 11.03125,
+ "stations": "Paez Survey,Jones Settlement,Heaviside Mines,Kirtley Installation,Lem Port,Smith's Progress"
+ },
+ {
+ "name": "Hreidmarda",
+ "pos_x": -38.59375,
+ "pos_y": -78.28125,
+ "pos_z": -140.3125,
+ "stations": "Wedge Mines"
+ },
+ {
+ "name": "Hreinja",
+ "pos_x": 36.75,
+ "pos_y": 88.15625,
+ "pos_z": -78.59375,
+ "stations": "Land Station"
+ },
+ {
+ "name": "Hreintania",
+ "pos_x": 86.53125,
+ "pos_y": 88.40625,
+ "pos_z": 49.28125,
+ "stations": "Hewish Orbital,Low Orbital,Gamow Enterprise,Buckland Keep,Kozeyev Gateway,Vela Orbital,Tarelkin Terminal,Mitchell Port,Elgin Gateway,Pytheas Gateway,Frechet Legacy"
+ },
+ {
+ "name": "Hreip",
+ "pos_x": -113.84375,
+ "pos_y": -78.9375,
+ "pos_z": 72.25,
+ "stations": "Onufrienko Installation,Onnes Settlement"
+ },
+ {
+ "name": "Hreipner",
+ "pos_x": -13.71875,
+ "pos_y": -139.40625,
+ "pos_z": 69.46875,
+ "stations": "Lowell Prospect,Shute Terminal,Valigursky Works"
+ },
+ {
+ "name": "Hrimfaxi",
+ "pos_x": 39.125,
+ "pos_y": -0.125,
+ "pos_z": -45.6875,
+ "stations": "Blackwell City,Covey Orbital,Filipchenko City,Aleksandrov Landing,Ahern Landing,Morris Prospect"
+ },
+ {
+ "name": "Hrisastshi",
+ "pos_x": -38.4375,
+ "pos_y": -28.78125,
+ "pos_z": 34.90625,
+ "stations": "Bounds Hub,Ivins Depot"
+ },
+ {
+ "name": "Hrisates",
+ "pos_x": 15.5,
+ "pos_y": -191,
+ "pos_z": 123.8125,
+ "stations": "Adelman Landing,Grzimek Penal colony"
+ },
+ {
+ "name": "Hrun",
+ "pos_x": 158.21875,
+ "pos_y": -11.625,
+ "pos_z": 25.46875,
+ "stations": "Poisson Mines,Hermite Point,Clark Landing"
+ },
+ {
+ "name": "Hrunapori",
+ "pos_x": 77.15625,
+ "pos_y": -60.28125,
+ "pos_z": -11.28125,
+ "stations": "Goryu Dock,Steiner Works,Galle Dock,Darboux Prospect,Homer City"
+ },
+ {
+ "name": "Hrunda",
+ "pos_x": -61.375,
+ "pos_y": 19.9375,
+ "pos_z": -99.625,
+ "stations": "Houssay Refinery"
+ },
+ {
+ "name": "Hrungubuju",
+ "pos_x": 70.75,
+ "pos_y": 60.75,
+ "pos_z": -18.25,
+ "stations": "Salam Orbital,Bacon Silo,Midgeley Landing"
+ },
+ {
+ "name": "Hruntia",
+ "pos_x": 64.09375,
+ "pos_y": -123.46875,
+ "pos_z": -15.6875,
+ "stations": "Shoemaker Port,Chandra Hub,Luyten Market,Naubakht Vision,Giraud Installation,Tedin Horizons,Albumasar Station,Shavyrin Keep,Baille Hub,Langley Terminal,al-Din al-Urdi Terminal,Krenkel Hub,Quetelet Station,Hadid Reach"
+ },
+ {
+ "name": "Hrymonada",
+ "pos_x": 98.59375,
+ "pos_y": -36.5625,
+ "pos_z": -39.625,
+ "stations": "Schilling Enterprise,Reynolds Park,Stableford Terminal"
+ },
+ {
+ "name": "Hsie",
+ "pos_x": -84.40625,
+ "pos_y": -16.6875,
+ "pos_z": -147.96875,
+ "stations": "Laue Prospect,Russo Observatory"
+ },
+ {
+ "name": "Hsien Baji",
+ "pos_x": -49.1875,
+ "pos_y": 20.21875,
+ "pos_z": -43.625,
+ "stations": "Stirling Platform,Strekalov Hangar"
+ },
+ {
+ "name": "Hsien Sham",
+ "pos_x": -26.84375,
+ "pos_y": -89.5625,
+ "pos_z": 123.75,
+ "stations": "Tito Station,McArthur Orbital,Levchenko Port,Hodgson Point"
+ },
+ {
+ "name": "Hsinga",
+ "pos_x": 10.59375,
+ "pos_y": -73.53125,
+ "pos_z": -41.96875,
+ "stations": "Russell Landing,Crampton Survey"
+ },
+ {
+ "name": "Hsini",
+ "pos_x": -107.84375,
+ "pos_y": 10.75,
+ "pos_z": -80.28125,
+ "stations": "Shaver Landing,Abel's Inheritance,Blaylock Landing"
+ },
+ {
+ "name": "Hsua Bumi",
+ "pos_x": 158.75,
+ "pos_y": -59.53125,
+ "pos_z": -4.375,
+ "stations": "Burnham Vision"
+ },
+ {
+ "name": "Hsuan Zu",
+ "pos_x": 114.3125,
+ "pos_y": -246.28125,
+ "pos_z": 110.09375,
+ "stations": "Blaauw Settlement,Nansen Survey"
+ },
+ {
+ "name": "Hsuang",
+ "pos_x": 100.125,
+ "pos_y": -143.34375,
+ "pos_z": 136.5625,
+ "stations": "Unsold Stop,Jones Terminal"
+ },
+ {
+ "name": "Hsuani",
+ "pos_x": -122.25,
+ "pos_y": -51.34375,
+ "pos_z": 119.0625,
+ "stations": "Napier Terminal,Norgay Dock,Adams Depot,Pordenone Vision"
+ },
+ {
+ "name": "Hsuanqueno",
+ "pos_x": -7.03125,
+ "pos_y": -117.25,
+ "pos_z": 89.09375,
+ "stations": "Grant Horizons,Jekhowsky City,Meech Orbital,Lem Installation"
+ },
+ {
+ "name": "Hu Jing Te",
+ "pos_x": 115.9375,
+ "pos_y": 37.96875,
+ "pos_z": -61.15625,
+ "stations": "Caidin Enterprise,Remec Station,Stasheff City,Quimper Station,Thompson Enterprise,Kier Station,Peirce Orbital,Clarke Port,Geston Port,Harding Reach,Darlton's Progress,Herreshoff Gateway,Tiedemann Exchange"
+ },
+ {
+ "name": "Hu Jini",
+ "pos_x": 139.125,
+ "pos_y": -22.09375,
+ "pos_z": 2.96875,
+ "stations": "Chandler Plant,Chwedyk Orbital,Webb Dock,King Dock,Markham Terminal"
+ },
+ {
+ "name": "Hu Jona",
+ "pos_x": -84,
+ "pos_y": -9.21875,
+ "pos_z": 3.21875,
+ "stations": "Russell Station,Zoline Station,Hyecho Terminal,Crown Dock,Crichton City,Rowley Terminal,Markov Arsenal,Kovalevskaya Dock,Pratchett Station,Lavoisier Enterprise,Roddenberry Orbital,Willis Escape,Schmitz Enterprise"
+ },
+ {
+ "name": "Hu Jun",
+ "pos_x": -62.09375,
+ "pos_y": -52.96875,
+ "pos_z": 106.3125,
+ "stations": "Zebrowski Settlement,Cartmill Freeport"
+ },
+ {
+ "name": "Hu Jung",
+ "pos_x": 26.375,
+ "pos_y": -90.75,
+ "pos_z": -63.1875,
+ "stations": "Harvey Dock"
+ },
+ {
+ "name": "Huacae",
+ "pos_x": 48.09375,
+ "pos_y": 75.9375,
+ "pos_z": 113.53125,
+ "stations": "Kozeyev Landing,Babcock Horizons,Lawrence Platform"
+ },
+ {
+ "name": "Huachipaeri",
+ "pos_x": 50.65625,
+ "pos_y": -0.15625,
+ "pos_z": -125.78125,
+ "stations": "Moresby Freeport,Baird Landing"
+ },
+ {
+ "name": "Huai Hait",
+ "pos_x": 151.71875,
+ "pos_y": -108.0625,
+ "pos_z": 30.15625,
+ "stations": "Mouchez Landing,Aaronson Base,Somayaji Port"
+ },
+ {
+ "name": "Huai Na",
+ "pos_x": 59.0625,
+ "pos_y": -198.6875,
+ "pos_z": 117.78125,
+ "stations": "Baille Vision,Fadlan Hub,Jung Platform"
+ },
+ {
+ "name": "Huaich",
+ "pos_x": -97.15625,
+ "pos_y": -96.28125,
+ "pos_z": 74.25,
+ "stations": "Schilling Dock,Lopez de Legazpi Prospect,Shaikh Platform"
+ },
+ {
+ "name": "Hual",
+ "pos_x": 22.1875,
+ "pos_y": 69.5625,
+ "pos_z": -6.4375,
+ "stations": "Younghusband Landing,Poncelet Terminal,Chern Installation"
+ },
+ {
+ "name": "Hualamsa",
+ "pos_x": 81.09375,
+ "pos_y": -170.65625,
+ "pos_z": 153.34375,
+ "stations": "Parkinson Base"
+ },
+ {
+ "name": "Hualarna",
+ "pos_x": 58.1875,
+ "pos_y": 54.53125,
+ "pos_z": 28,
+ "stations": "Gilliland Prospect,Julian Port,Reed City,Filter's Inheritance,Foda Plant,Berezovoy Platform"
+ },
+ {
+ "name": "Hualm",
+ "pos_x": 109.375,
+ "pos_y": 4.0625,
+ "pos_z": 69.78125,
+ "stations": "Fernandes de Queiros Plant,Walker Installation,Russo Hangar,Ashton Landing"
+ },
+ {
+ "name": "Huambisa",
+ "pos_x": -128.5,
+ "pos_y": -24.03125,
+ "pos_z": -18.96875,
+ "stations": "Tarski Point,Kingsmill Colony"
+ },
+ {
+ "name": "Huan Guang",
+ "pos_x": -43.4375,
+ "pos_y": -99.28125,
+ "pos_z": 115,
+ "stations": "Anthony Landing"
+ },
+ {
+ "name": "Huan Nu",
+ "pos_x": 180.15625,
+ "pos_y": -108.46875,
+ "pos_z": 34.90625,
+ "stations": "Truly Port,Sheepshanks Orbital,Kawasato Hub,Harding Beacon"
+ },
+ {
+ "name": "Huandiga",
+ "pos_x": -72.59375,
+ "pos_y": 27.6875,
+ "pos_z": -104.28125,
+ "stations": "Edwards Works,Vaugh Mines,Berners-Lee Terminal"
+ },
+ {
+ "name": "Huandja",
+ "pos_x": -92.375,
+ "pos_y": 2.5625,
+ "pos_z": 92.15625,
+ "stations": "Crampton Market,Irvin Station,Sullivan Enterprise,Malpighi Terminal"
+ },
+ {
+ "name": "Huang Gun",
+ "pos_x": 147.3125,
+ "pos_y": -180,
+ "pos_z": 77.875,
+ "stations": "Pittendreigh City"
+ },
+ {
+ "name": "Huang Yai",
+ "pos_x": 125.5,
+ "pos_y": 7.09375,
+ "pos_z": 0.3125,
+ "stations": "Sullivan Ring,Junlong Terminal,Stableford Landing,Hopkins Vision,Jeury Arsenal,Bates Oasis"
+ },
+ {
+ "name": "Huangais",
+ "pos_x": 72.53125,
+ "pos_y": -12.03125,
+ "pos_z": 10.71875,
+ "stations": "Blackwell Station,Walter Orbital,Cooper Orbital,Fairbairn Prospect,Napier Vista,Rotsler Enterprise,Fujimori Station,Fermi Ring,Burnell Forum"
+ },
+ {
+ "name": "Huano",
+ "pos_x": 80.09375,
+ "pos_y": -92.0625,
+ "pos_z": -96.59375,
+ "stations": "White Enterprise,Maybury Port,Ashton Terminal"
+ },
+ {
+ "name": "Huaorani",
+ "pos_x": -65.375,
+ "pos_y": 70,
+ "pos_z": -84.3125,
+ "stations": "Quaglia Arena"
+ },
+ {
+ "name": "Huave",
+ "pos_x": -40.25,
+ "pos_y": -139.1875,
+ "pos_z": 16.25,
+ "stations": "Lemaitre Holdings,DiFate Landing,Mead Port"
+ },
+ {
+ "name": "Huaveri",
+ "pos_x": 113.53125,
+ "pos_y": 5.375,
+ "pos_z": 63.65625,
+ "stations": "Watson Orbital"
+ },
+ {
+ "name": "Huh",
+ "pos_x": -26.875,
+ "pos_y": -34.375,
+ "pos_z": 37.1875,
+ "stations": "Komarov Landing,Patsayev Laboratory,Moisuc Refinery"
+ },
+ {
+ "name": "Huiche",
+ "pos_x": -50.6875,
+ "pos_y": -18.59375,
+ "pos_z": -57.625,
+ "stations": "Garriott Hub,Kizim Station,Naddoddur Observatory,Levi-Strauss Gateway,Nikitin Base"
+ },
+ {
+ "name": "Huichi",
+ "pos_x": -15.9375,
+ "pos_y": -74.75,
+ "pos_z": 18.21875,
+ "stations": "Collins Station,Johnson Orbital"
+ },
+ {
+ "name": "Huichis",
+ "pos_x": -58.75,
+ "pos_y": -162.90625,
+ "pos_z": -15.21875,
+ "stations": "Guerrero's Pride"
+ },
+ {
+ "name": "Huichu",
+ "pos_x": 50.59375,
+ "pos_y": -146.84375,
+ "pos_z": 87.09375,
+ "stations": "Grunsfeld Hub,Gehrels Colony,Chertok Beacon"
+ },
+ {
+ "name": "Huile",
+ "pos_x": -97.40625,
+ "pos_y": 75,
+ "pos_z": -83.84375,
+ "stations": "Ellison Base,Boole Dock"
+ },
+ {
+ "name": "Huilliche",
+ "pos_x": -79.875,
+ "pos_y": -13.5625,
+ "pos_z": 48.78125,
+ "stations": "Piercy Gateway,Lee Enterprise"
+ },
+ {
+ "name": "Huldr",
+ "pos_x": -8.34375,
+ "pos_y": 149.125,
+ "pos_z": 76.1875,
+ "stations": "Flade Dock,Shatalov Landing,Oersted Terminal,Teng-hui Prospect"
+ },
+ {
+ "name": "Huma",
+ "pos_x": 44.0625,
+ "pos_y": -131.90625,
+ "pos_z": -23.15625,
+ "stations": "Whelan City,McQuarrie Port,Bahcall Hub,Schwarzschild Station"
+ },
+ {
+ "name": "Humana",
+ "pos_x": 116.75,
+ "pos_y": -245.4375,
+ "pos_z": 40.3125,
+ "stations": "Qian Depot,Haro Hub,Maskelyne Outpost,Brothers Terminal"
+ },
+ {
+ "name": "Humania",
+ "pos_x": -21.75,
+ "pos_y": -22.40625,
+ "pos_z": 106.75,
+ "stations": "Piserchia Freeport,Crumey Landing,Henslow Installation,MacVicar Camp"
+ },
+ {
+ "name": "Humanicnii",
+ "pos_x": 185.0625,
+ "pos_y": -158.09375,
+ "pos_z": 38.1875,
+ "stations": "Fraknoi Station,Shavyrin Landing,Plucker Relay"
+ },
+ {
+ "name": "Humarala",
+ "pos_x": -138.03125,
+ "pos_y": -62.25,
+ "pos_z": -12.4375,
+ "stations": "Dunyach Platform"
+ },
+ {
+ "name": "Humatsu",
+ "pos_x": 46.5625,
+ "pos_y": -171.09375,
+ "pos_z": -61.03125,
+ "stations": "Longomontanus Beacon"
+ },
+ {
+ "name": "Hun Ahaw",
+ "pos_x": 121.125,
+ "pos_y": 16.3125,
+ "pos_z": -2.96875,
+ "stations": "Bessemer Escape,Lorentz Terminal,Oersted Port"
+ },
+ {
+ "name": "Hun Ahayuse",
+ "pos_x": -15.40625,
+ "pos_y": 4.8125,
+ "pos_z": 75.625,
+ "stations": "Arkwright Gateway,Hopkins Gateway,Newman Ring,Vercors Enterprise"
+ },
+ {
+ "name": "Hun Aphuill",
+ "pos_x": 49.09375,
+ "pos_y": -152.3125,
+ "pos_z": -101.625,
+ "stations": "Fox Dock,Morrison's Progress"
+ },
+ {
+ "name": "Hun Binja",
+ "pos_x": -8.03125,
+ "pos_y": 129.15625,
+ "pos_z": -80.1875,
+ "stations": "Rae Port"
+ },
+ {
+ "name": "Hun Chami",
+ "pos_x": -63.0625,
+ "pos_y": 19.09375,
+ "pos_z": -116.40625,
+ "stations": "Navigator Orbital,Kregel Relay"
+ },
+ {
+ "name": "Hun Chians",
+ "pos_x": 64.5625,
+ "pos_y": -110.78125,
+ "pos_z": 71.5625,
+ "stations": "Parkinson Vision,Murakami Settlement,Vaucouleurs Observatory"
+ },
+ {
+ "name": "Hun Chonses",
+ "pos_x": 9.125,
+ "pos_y": -3.71875,
+ "pos_z": 80.46875,
+ "stations": "Al-Khalili Hub,Stafford Station,McDivitt Dock,Morrow Vision"
+ },
+ {
+ "name": "Hun Cruac",
+ "pos_x": 189.53125,
+ "pos_y": -114.9375,
+ "pos_z": 15.09375,
+ "stations": "Bauschinger Station,Weill Orbital,Dalgarno Gateway,Ricci's Folly"
+ },
+ {
+ "name": "Hun Cruacoc",
+ "pos_x": -84.375,
+ "pos_y": 74.625,
+ "pos_z": 85,
+ "stations": "Fabian Port,Akiyama Station,Carter Installation,Bakewell Station,Patsayev Orbital,Fairbairn Orbital,Blaha Orbital,McCandless Orbital,Oxley Relay,Chamitoff Depot,Malocello Holdings"
+ },
+ {
+ "name": "Hun Du",
+ "pos_x": -6.25,
+ "pos_y": -190.21875,
+ "pos_z": 98.96875,
+ "stations": "Drake Settlement,Kulin Mine,Miyasaka Platform"
+ },
+ {
+ "name": "Hun Dun",
+ "pos_x": 116.09375,
+ "pos_y": -56.96875,
+ "pos_z": 81.84375,
+ "stations": "McCool Vision"
+ },
+ {
+ "name": "Hun Hi",
+ "pos_x": 142.09375,
+ "pos_y": -150.34375,
+ "pos_z": 48.8125,
+ "stations": "Mobius Outpost"
+ },
+ {
+ "name": "Hun Hsinas",
+ "pos_x": 145.5625,
+ "pos_y": 55.65625,
+ "pos_z": -46.9375,
+ "stations": "Merchiston Beacon,Stiegler Depot,Banach Survey"
+ },
+ {
+ "name": "Hun Nal Yeh",
+ "pos_x": 75.71875,
+ "pos_y": 58.875,
+ "pos_z": 60.375,
+ "stations": "Vesalius Colony"
+ },
+ {
+ "name": "Hun Nef",
+ "pos_x": 17.46875,
+ "pos_y": -56.625,
+ "pos_z": 137.90625,
+ "stations": "Armstrong Landing"
+ },
+ {
+ "name": "Hun Nighu",
+ "pos_x": 136.5,
+ "pos_y": -75.59375,
+ "pos_z": 103.28125,
+ "stations": "Dyson Works,Iwamoto Legacy"
+ },
+ {
+ "name": "Hun Nik",
+ "pos_x": 155.6875,
+ "pos_y": 107.125,
+ "pos_z": -78.65625,
+ "stations": "Phillifent Freeport,Muhammad Ibn Battuta Holdings"
+ },
+ {
+ "name": "Hun Nongga",
+ "pos_x": 98.8125,
+ "pos_y": -84.71875,
+ "pos_z": 92,
+ "stations": "Barlowe Terminal"
+ },
+ {
+ "name": "Hun Nu",
+ "pos_x": -76.03125,
+ "pos_y": 50.1875,
+ "pos_z": -110.28125,
+ "stations": "Forstchen Station,Simonyi Base,Beatty Plant,Samuda Orbital,Griffin Dock"
+ },
+ {
+ "name": "Hun Pef",
+ "pos_x": 158.21875,
+ "pos_y": -107.75,
+ "pos_z": 39.65625,
+ "stations": "Thesiger Refinery,Flaugergues Dock,Schmidt Platform"
+ },
+ {
+ "name": "Hun Pi",
+ "pos_x": -120.4375,
+ "pos_y": -59.53125,
+ "pos_z": -84.875,
+ "stations": "Babbage Colony"
+ },
+ {
+ "name": "Hun Puggsa",
+ "pos_x": -143.34375,
+ "pos_y": 76.0625,
+ "pos_z": -40.96875,
+ "stations": "Nixon Beacon,Chandler Beacon,Patterson Installation"
+ },
+ {
+ "name": "Hun Pulan",
+ "pos_x": 7.21875,
+ "pos_y": -80.28125,
+ "pos_z": -109.40625,
+ "stations": "Treshchov Station,Fung Hub,Bain Forum,Sarafanov Port"
+ },
+ {
+ "name": "Hun Ti",
+ "pos_x": -62.96875,
+ "pos_y": 41.96875,
+ "pos_z": 100.96875,
+ "stations": "Gooch Point,Volta Camp"
+ },
+ {
+ "name": "Hun Tsach",
+ "pos_x": 12.09375,
+ "pos_y": -13.375,
+ "pos_z": 143.78125,
+ "stations": "Eanes Survey,Tanaka Camp"
+ },
+ {
+ "name": "Hun Wu",
+ "pos_x": 88.125,
+ "pos_y": 53.78125,
+ "pos_z": -103.59375,
+ "stations": "Dowling Asylum,Tull's Progress"
+ },
+ {
+ "name": "Hunaharten",
+ "pos_x": 2.1875,
+ "pos_y": -40.09375,
+ "pos_z": 82.53125,
+ "stations": "Olsen Terminal,Reiter Keep,Brust Beacon"
+ },
+ {
+ "name": "Hunahati",
+ "pos_x": 191.125,
+ "pos_y": -160.5625,
+ "pos_z": 11.65625,
+ "stations": "van de Sande Bakhuyzen Mine,Macedo Installation"
+ },
+ {
+ "name": "Hunahau",
+ "pos_x": 46.625,
+ "pos_y": 23.96875,
+ "pos_z": -58.15625,
+ "stations": "Vogel Orbital"
+ },
+ {
+ "name": "Hunapel",
+ "pos_x": 160.3125,
+ "pos_y": -86.0625,
+ "pos_z": 40.65625,
+ "stations": "Russell Ring,Mitchell Camp,Yu Base,Parker Station,Calatrava Installation"
+ },
+ {
+ "name": "Hunapep",
+ "pos_x": 85.1875,
+ "pos_y": -52.25,
+ "pos_z": 119.34375,
+ "stations": "Korlevic Mines"
+ },
+ {
+ "name": "Hunapese",
+ "pos_x": 42.625,
+ "pos_y": -206.0625,
+ "pos_z": 3.5625,
+ "stations": "Schulhof Stop"
+ },
+ {
+ "name": "Hunapiti",
+ "pos_x": -43.0625,
+ "pos_y": -73.6875,
+ "pos_z": 125.4375,
+ "stations": "Evans Orbital,Birmingham Installation,Bunch Gateway"
+ },
+ {
+ "name": "Hunbina",
+ "pos_x": 135.5625,
+ "pos_y": 71.25,
+ "pos_z": 15.90625,
+ "stations": "Muhammad Ibn Battuta Horizons,Chalker Camp,Hoyle Point"
+ },
+ {
+ "name": "Hunbinja",
+ "pos_x": -100.03125,
+ "pos_y": 61.71875,
+ "pos_z": 58.4375,
+ "stations": "Morgan Hub,Apt Gateway,Hoshide Dock,Cook Keep"
+ },
+ {
+ "name": "Hunbudji",
+ "pos_x": 171.09375,
+ "pos_y": -73.09375,
+ "pos_z": 6.125,
+ "stations": "Burnham Port,Hollander Landing"
+ },
+ {
+ "name": "Hunbul",
+ "pos_x": 20.375,
+ "pos_y": 78.59375,
+ "pos_z": -63.0625,
+ "stations": "Hartlib Orbital,Kizim Gateway"
+ },
+ {
+ "name": "Hunburra",
+ "pos_x": -31.84375,
+ "pos_y": 45.75,
+ "pos_z": 139.40625,
+ "stations": "Lawrence Holdings,Still Survey"
+ },
+ {
+ "name": "Huncetae",
+ "pos_x": -34.03125,
+ "pos_y": -213.875,
+ "pos_z": 106.59375,
+ "stations": "Langley Relay,Shimizu's Progress,Galouye Enterprise"
+ },
+ {
+ "name": "Hunchis",
+ "pos_x": 180.15625,
+ "pos_y": -159.21875,
+ "pos_z": 21.125,
+ "stations": "Ayton Innes Station,Goodricke Survey,Bowell Depot"
+ },
+ {
+ "name": "Hunchosgan",
+ "pos_x": -22.375,
+ "pos_y": -217.15625,
+ "pos_z": 39.75,
+ "stations": "von Zach Enterprise,Palisa Installation,Mil Terminal,Haro Station,Saunders Base"
+ },
+ {
+ "name": "Hunchosim",
+ "pos_x": -2.75,
+ "pos_y": 55.3125,
+ "pos_z": -100.5625,
+ "stations": "Roentgen Landing,Bloch Settlement,Liska Terminal"
+ },
+ {
+ "name": "Hungalun",
+ "pos_x": -132.90625,
+ "pos_y": 92.8125,
+ "pos_z": 40.96875,
+ "stations": "Lewis Platform,Dayuan Dock,Kotzebue Base"
+ },
+ {
+ "name": "Hungua",
+ "pos_x": -114.78125,
+ "pos_y": 113.90625,
+ "pos_z": 79.25,
+ "stations": "Buckland Survey"
+ },
+ {
+ "name": "Hunharecomi",
+ "pos_x": 148.59375,
+ "pos_y": -79.15625,
+ "pos_z": 99.28125,
+ "stations": "Eisinga Orbital,Bereznyak Vision"
+ },
+ {
+ "name": "Hunhau",
+ "pos_x": -43.375,
+ "pos_y": -152.59375,
+ "pos_z": 57.71875,
+ "stations": "Hay Hub,Michell Station,Flynn Prospect,Johri Penal colony"
+ },
+ {
+ "name": "Huntan",
+ "pos_x": -40.5,
+ "pos_y": -102.15625,
+ "pos_z": -60.875,
+ "stations": "Clark Dock,Hillary Vision,Peral Landing,Adams Point"
+ },
+ {
+ "name": "Hunte",
+ "pos_x": 29.65625,
+ "pos_y": -150.90625,
+ "pos_z": 34.9375,
+ "stations": "Thierree Station"
+ },
+ {
+ "name": "Huntics",
+ "pos_x": 158.28125,
+ "pos_y": -123.78125,
+ "pos_z": -0.03125,
+ "stations": "Bolden Landing,Dittmar Hub,Bartini Gateway,Noon Vision,Brooks Beacon"
+ },
+ {
+ "name": "Hunza",
+ "pos_x": 132.96875,
+ "pos_y": -70.21875,
+ "pos_z": 66.15625,
+ "stations": "Frazetta Port,Kidinnu Hub,Fu Hub,Schlesinger Terminal"
+ },
+ {
+ "name": "Hunzi",
+ "pos_x": 132,
+ "pos_y": -180.03125,
+ "pos_z": 102.8125,
+ "stations": "Fischer Vision,Barbosa Survey"
+ },
+ {
+ "name": "Hunzicnal",
+ "pos_x": 104.8125,
+ "pos_y": -64.125,
+ "pos_z": 85.8125,
+ "stations": "Eddington Vision,Hampson Mines,He Base,Vaucouleurs Platform"
+ },
+ {
+ "name": "Huokang",
+ "pos_x": -12.1875,
+ "pos_y": 35.46875,
+ "pos_z": -25.28125,
+ "stations": "Steiner City,Markov Oasis,Berezin Dock"
+ },
+ {
+ "name": "Hupako",
+ "pos_x": -79.9375,
+ "pos_y": -45.375,
+ "pos_z": -16.71875,
+ "stations": "Gresley Base,Sharma Survey,Rontgen Refinery"
+ },
+ {
+ "name": "Hupan",
+ "pos_x": -40.15625,
+ "pos_y": -61.4375,
+ "pos_z": 80.25,
+ "stations": "Schmitt Hub,Antonelli Depot"
+ },
+ {
+ "name": "Hupang",
+ "pos_x": 94.84375,
+ "pos_y": -77.625,
+ "pos_z": -116.03125,
+ "stations": "Davy Beacon,Brash's Folly"
+ },
+ {
+ "name": "Hupanga",
+ "pos_x": 21.96875,
+ "pos_y": -105.90625,
+ "pos_z": 156.4375,
+ "stations": "Herschel Survey,Apianus Colony,Lie Vision"
+ },
+ {
+ "name": "Huruk",
+ "pos_x": -4.78125,
+ "pos_y": -183.34375,
+ "pos_z": -43.125,
+ "stations": "Harvey-Smith Holdings"
+ },
+ {
+ "name": "Hurukuntak",
+ "pos_x": 55.03125,
+ "pos_y": -24.9375,
+ "pos_z": 116.75,
+ "stations": "Bauschinger Terminal,Dickson Point,Coelho Beacon,Bullialdus City,South City"
+ },
+ {
+ "name": "Hurusalki",
+ "pos_x": -104.59375,
+ "pos_y": 93.625,
+ "pos_z": -23.5625,
+ "stations": "Kessel Terminal,Stephens Station,Cardano City,Blish Installation,Flynn Station,Wilhelm Settlement,Cousin Point,Wisniewski-Snerg Orbital"
+ },
+ {
+ "name": "Huveang De",
+ "pos_x": -40.71875,
+ "pos_y": -22.84375,
+ "pos_z": -114.75,
+ "stations": "Galiano Enterprise,Haignere Port"
+ },
+ {
+ "name": "Hyades Sector AB-W b2-2",
+ "pos_x": -91.65625,
+ "pos_y": -62.53125,
+ "pos_z": -224.5,
+ "stations": "PRE Logistics Support Delta,Exodus Point,Rescue Ship - Exodus Point"
+ },
+ {
+ "name": "Hyades Sector AV-O b6-1",
+ "pos_x": 61.40625,
+ "pos_y": -72.6875,
+ "pos_z": -139.5625,
+ "stations": "Shalatula Installation"
+ },
+ {
+ "name": "Hyades Sector AV-O b6-5",
+ "pos_x": 71.25,
+ "pos_y": -73.5,
+ "pos_z": -138.65625,
+ "stations": "Timofeyevich's Inheritance,Scheerbart Horizons"
+ },
+ {
+ "name": "Hyades Sector BB-W c2-10",
+ "pos_x": 13.8125,
+ "pos_y": 76.1875,
+ "pos_z": -147,
+ "stations": "Chwedyk Relay"
+ },
+ {
+ "name": "Hyades Sector BG-X d1-61",
+ "pos_x": -0.875,
+ "pos_y": 77.5625,
+ "pos_z": -156.5625,
+ "stations": "Shirley Horizons"
+ },
+ {
+ "name": "Hyades Sector CB-W c2-1",
+ "pos_x": 49.65625,
+ "pos_y": 56.90625,
+ "pos_z": -169.03125,
+ "stations": "Crumey Enterprise"
+ },
+ {
+ "name": "Hyades Sector DB-X d1-115",
+ "pos_x": -40.8125,
+ "pos_y": -0.71875,
+ "pos_z": -183.5625,
+ "stations": "Cobb Settlement"
+ },
+ {
+ "name": "Hyades Sector DR-V c2-13",
+ "pos_x": -92.46875,
+ "pos_y": 2.21875,
+ "pos_z": -176.875,
+ "stations": "Grassmann Beacon"
+ },
+ {
+ "name": "Hyades Sector DR-V c2-23",
+ "pos_x": -104.625,
+ "pos_y": -0.8125,
+ "pos_z": -151.90625,
+ "stations": "Asire Base"
+ },
+ {
+ "name": "Hyades Sector DR-V c2-3",
+ "pos_x": -82.625,
+ "pos_y": 6.6875,
+ "pos_z": -180.15625,
+ "stations": "Lee Enterprise"
+ },
+ {
+ "name": "Hyades Sector EB-X d1-104",
+ "pos_x": 25.4375,
+ "pos_y": -7.375,
+ "pos_z": -156.21875,
+ "stations": "Cousteau Vision"
+ },
+ {
+ "name": "Hyades Sector EB-X d1-106",
+ "pos_x": 15.9375,
+ "pos_y": -8.625,
+ "pos_z": -150.71875,
+ "stations": "Kidman Landing"
+ },
+ {
+ "name": "Hyades Sector EB-X d1-110",
+ "pos_x": 39.53125,
+ "pos_y": -7.4375,
+ "pos_z": -115,
+ "stations": "Fernandes Terminal,Irvin's Progress"
+ },
+ {
+ "name": "Hyades Sector EB-X d1-112",
+ "pos_x": 71.96875,
+ "pos_y": -11.9375,
+ "pos_z": -158.34375,
+ "stations": "Reed's Inheritance"
+ },
+ {
+ "name": "Hyades Sector EB-X d1-54",
+ "pos_x": 69.375,
+ "pos_y": 12.90625,
+ "pos_z": -168.8125,
+ "stations": "Harper Enterprise"
+ },
+ {
+ "name": "Hyades Sector EB-X d1-56",
+ "pos_x": 25.15625,
+ "pos_y": 40.96875,
+ "pos_z": -175.90625,
+ "stations": "Dunyach Relay"
+ },
+ {
+ "name": "Hyades Sector ER-V c2-20",
+ "pos_x": -33.4375,
+ "pos_y": -4.53125,
+ "pos_z": -174.15625,
+ "stations": "Spielberg Installation,Gromov Holdings"
+ },
+ {
+ "name": "Hyades Sector FB-X d1-41",
+ "pos_x": 99.125,
+ "pos_y": -8.9375,
+ "pos_z": -131.875,
+ "stations": "Palmer Installation"
+ },
+ {
+ "name": "Hyades Sector FR-V c2-13",
+ "pos_x": -20.40625,
+ "pos_y": 10.46875,
+ "pos_z": -162.4375,
+ "stations": "Moore Stop"
+ },
+ {
+ "name": "Hyades Sector FW-V c2-20",
+ "pos_x": 63.96875,
+ "pos_y": 44.03125,
+ "pos_z": -168.71875,
+ "stations": "Burgess' Inheritance,Kolmogorov Landing"
+ },
+ {
+ "name": "Hyades Sector FW-V c2-6",
+ "pos_x": 94.90625,
+ "pos_y": 45.5,
+ "pos_z": -149.21875,
+ "stations": "Chios Arsenal"
+ },
+ {
+ "name": "Hyades Sector FW-V c2-8",
+ "pos_x": 64.34375,
+ "pos_y": 44.8125,
+ "pos_z": -175.15625,
+ "stations": "Davidson Landing"
+ },
+ {
+ "name": "Hyades Sector GH-V d2-135",
+ "pos_x": -80.625,
+ "pos_y": 0.625,
+ "pos_z": -79.96875,
+ "stations": "Ahern Settlement,Blaylock Point"
+ },
+ {
+ "name": "Hyades Sector GH-V d2-97",
+ "pos_x": -121.21875,
+ "pos_y": -17.375,
+ "pos_z": -85.21875,
+ "stations": "Mallory Arsenal,Johnson Enterprise"
+ },
+ {
+ "name": "Hyades Sector GR-V c2-14",
+ "pos_x": 47.75,
+ "pos_y": 1.1875,
+ "pos_z": -152.59375,
+ "stations": "la Cosa Installation"
+ },
+ {
+ "name": "Hyades Sector GX-T c3-18",
+ "pos_x": -105.71875,
+ "pos_y": 5,
+ "pos_z": -127.4375,
+ "stations": "Taine Survey"
+ },
+ {
+ "name": "Hyades Sector GX-T c3-2",
+ "pos_x": -137.1875,
+ "pos_y": -22.34375,
+ "pos_z": -121.21875,
+ "stations": "Clair Horizons"
+ },
+ {
+ "name": "Hyades Sector GX-T c3-25",
+ "pos_x": -128.40625,
+ "pos_y": -13.5,
+ "pos_z": -133.9375,
+ "stations": "Ore Lab"
+ },
+ {
+ "name": "Hyades Sector GX-T c3-6",
+ "pos_x": -119.46875,
+ "pos_y": -3.625,
+ "pos_z": -110.59375,
+ "stations": "Scully-Power Horizons,Thompson Penal colony"
+ },
+ {
+ "name": "Hyades Sector HC-U c3-10",
+ "pos_x": 12.65625,
+ "pos_y": 28.84375,
+ "pos_z": -137,
+ "stations": "Pohl Beacon,Payson Vision,Springer Terminal"
+ },
+ {
+ "name": "Hyades Sector HI-R b5-0",
+ "pos_x": -81.03125,
+ "pos_y": -1.71875,
+ "pos_z": -164.8125,
+ "stations": "Bailey Plant"
+ },
+ {
+ "name": "Hyades Sector HR-V c2-9",
+ "pos_x": 70,
+ "pos_y": 7.9375,
+ "pos_z": -179.875,
+ "stations": "Kube-McDowell Horizons"
+ },
+ {
+ "name": "Hyades Sector HR-W d1-59",
+ "pos_x": -6.75,
+ "pos_y": -123.03125,
+ "pos_z": -173.90625,
+ "stations": "Campbell Base,Bottego Base,Soper Installation"
+ },
+ {
+ "name": "Hyades Sector HR-W d1-61",
+ "pos_x": 14.3125,
+ "pos_y": -115,
+ "pos_z": -114.625,
+ "stations": "Silva Depot,Dias Relay"
+ },
+ {
+ "name": "Hyades Sector HS-R b5-0",
+ "pos_x": -1.3125,
+ "pos_y": 36.40625,
+ "pos_z": -163.53125,
+ "stations": "Love's Folly,Boe Depot"
+ },
+ {
+ "name": "Hyades Sector HW-W d1-52",
+ "pos_x": 100.65625,
+ "pos_y": -81.125,
+ "pos_z": -128.75,
+ "stations": "Blair Asylum"
+ },
+ {
+ "name": "Hyades Sector HW-W d1-64",
+ "pos_x": 110.09375,
+ "pos_y": -41,
+ "pos_z": -138.09375,
+ "stations": "Gurragchaa Horizons"
+ },
+ {
+ "name": "Hyades Sector IC-K b9-4",
+ "pos_x": 112.84375,
+ "pos_y": -13.5,
+ "pos_z": -83.6875,
+ "stations": "Bachman Survey"
+ },
+ {
+ "name": "Hyades Sector IC-U c3-11",
+ "pos_x": 46.9375,
+ "pos_y": 17.90625,
+ "pos_z": -121.6875,
+ "stations": "Malcolm Bastion,Garneau's Claim"
+ },
+ {
+ "name": "Hyades Sector IC-U c3-9",
+ "pos_x": 42.71875,
+ "pos_y": 21.71875,
+ "pos_z": -124.65625,
+ "stations": "Hale Silo,Roberts Terminal,Pavlov Settlement,Kepler Holdings"
+ },
+ {
+ "name": "Hyades Sector IH-V d2-130",
+ "pos_x": 88.34375,
+ "pos_y": 29.5625,
+ "pos_z": -93.375,
+ "stations": "Crook Beacon"
+ },
+ {
+ "name": "Hyades Sector IR-W d1-59",
+ "pos_x": 50.46875,
+ "pos_y": -147.375,
+ "pos_z": -140.5625,
+ "stations": "Davidson Base,Tarentum Laboratory"
+ },
+ {
+ "name": "Hyades Sector IR-W d1-69",
+ "pos_x": 67.0625,
+ "pos_y": -154,
+ "pos_z": -120.28125,
+ "stations": "Bottego Landing"
+ },
+ {
+ "name": "Hyades Sector IR-W d1-82",
+ "pos_x": 52.1875,
+ "pos_y": -164.375,
+ "pos_z": -131.6875,
+ "stations": "Rothfuss Vision"
+ },
+ {
+ "name": "Hyades Sector JH-V c2-15",
+ "pos_x": 14.625,
+ "pos_y": -94.6875,
+ "pos_z": -155.0625,
+ "stations": "Kessel's Inheritance"
+ },
+ {
+ "name": "Hyades Sector JI-R b5-2",
+ "pos_x": -37.59375,
+ "pos_y": -0.125,
+ "pos_z": -163.5625,
+ "stations": "Antonio de Andrade Enterprise,Heyerdahl Base"
+ },
+ {
+ "name": "Hyades Sector JM-V c2-4",
+ "pos_x": 78.375,
+ "pos_y": -62.78125,
+ "pos_z": -163.6875,
+ "stations": "Brillant's Inheritance,Platt Enterprise"
+ },
+ {
+ "name": "Hyades Sector JM-V c2-5",
+ "pos_x": 75.90625,
+ "pos_y": -59.1875,
+ "pos_z": -164.78125,
+ "stations": "Foden Relay,Raleigh Observatory"
+ },
+ {
+ "name": "Hyades Sector JO-P b6-3",
+ "pos_x": -122.21875,
+ "pos_y": 11.65625,
+ "pos_z": -142.09375,
+ "stations": "Flynn Reformatory"
+ },
+ {
+ "name": "Hyades Sector KC-L b8-4",
+ "pos_x": 62.375,
+ "pos_y": -103.25,
+ "pos_z": -96.03125,
+ "stations": "Kress Relay,Blair Works"
+ },
+ {
+ "name": "Hyades Sector KC-U c3-8",
+ "pos_x": 115.375,
+ "pos_y": 27.375,
+ "pos_z": -126.5,
+ "stations": "Deluc Landing"
+ },
+ {
+ "name": "Hyades Sector KD-S c4-16",
+ "pos_x": -118.5,
+ "pos_y": -10.34375,
+ "pos_z": -97.75,
+ "stations": "Okorafor Terminal,Nasmyth Installation"
+ },
+ {
+ "name": "Hyades Sector KD-S c4-6",
+ "pos_x": -130.875,
+ "pos_y": -23.40625,
+ "pos_z": -100.78125,
+ "stations": "Bose Penal colony"
+ },
+ {
+ "name": "Hyades Sector KH-V c2-12",
+ "pos_x": 21.8125,
+ "pos_y": -71.625,
+ "pos_z": -156.125,
+ "stations": "Shiras Relay"
+ },
+ {
+ "name": "Hyades Sector KH-V c2-14",
+ "pos_x": 35.625,
+ "pos_y": -66.8125,
+ "pos_z": -158.78125,
+ "stations": "Manning Penal colony"
+ },
+ {
+ "name": "Hyades Sector KH-V c2-16",
+ "pos_x": 40.1875,
+ "pos_y": -75.71875,
+ "pos_z": -154.125,
+ "stations": "Viete Silo"
+ },
+ {
+ "name": "Hyades Sector KH-V c2-18",
+ "pos_x": 47.125,
+ "pos_y": -87.46875,
+ "pos_z": -151.25,
+ "stations": "al-Haytham Lab"
+ },
+ {
+ "name": "Hyades Sector KS-R b5-0",
+ "pos_x": 55.09375,
+ "pos_y": 38.0625,
+ "pos_z": -162.9375,
+ "stations": "Weyl Base"
+ },
+ {
+ "name": "Hyades Sector LC-L b8-0",
+ "pos_x": 93.71875,
+ "pos_y": -103.625,
+ "pos_z": -85.9375,
+ "stations": "The Fountain of Penance"
+ },
+ {
+ "name": "Hyades Sector LD-Q b6-1",
+ "pos_x": 48.28125,
+ "pos_y": 72.125,
+ "pos_z": -127.40625,
+ "stations": "Anderson Installation,Kandel Prospect"
+ },
+ {
+ "name": "Hyades Sector LD-R b5-1",
+ "pos_x": -31.28125,
+ "pos_y": -6.375,
+ "pos_z": -154.15625,
+ "stations": "Doctorow Vision"
+ },
+ {
+ "name": "Hyades Sector LD-S c4-28",
+ "pos_x": -86.34375,
+ "pos_y": 1.40625,
+ "pos_z": -80.5625,
+ "stations": "Britnev Landing,Daniel Terminal,Hand Bastion,Walters' Progress"
+ },
+ {
+ "name": "Hyades Sector LH-L b8-3",
+ "pos_x": 125.78125,
+ "pos_y": -65.28125,
+ "pos_z": -96.9375,
+ "stations": "Herodotus Penal colony"
+ },
+ {
+ "name": "Hyades Sector LN-R b5-4",
+ "pos_x": 53.8125,
+ "pos_y": 33.8125,
+ "pos_z": -160.46875,
+ "stations": "Lerman Barracks"
+ },
+ {
+ "name": "Hyades Sector LX-T c3-28",
+ "pos_x": 58.09375,
+ "pos_y": -18.1875,
+ "pos_z": -123.8125,
+ "stations": "Galois' Folly,Anderson Installation"
+ },
+ {
+ "name": "Hyades Sector LX-U d2-76",
+ "pos_x": -25.625,
+ "pos_y": -160.09375,
+ "pos_z": -94.40625,
+ "stations": "Gunn Base"
+ },
+ {
+ "name": "Hyades Sector LX-U d2-84",
+ "pos_x": 4.5625,
+ "pos_y": -150.4375,
+ "pos_z": -100.96875,
+ "stations": "Gromov Plant,Flinders Penal colony"
+ },
+ {
+ "name": "Hyades Sector MD-R b5-0",
+ "pos_x": -13.34375,
+ "pos_y": -16.25,
+ "pos_z": -146.25,
+ "stations": "Diophantus Silo,Lee Base"
+ },
+ {
+ "name": "Hyades Sector MD-R b5-4",
+ "pos_x": -11.34375,
+ "pos_y": -13.8125,
+ "pos_z": -160.6875,
+ "stations": "Stephenson Works,Haldeman Vision"
+ },
+ {
+ "name": "Hyades Sector MX-T c3-15",
+ "pos_x": 111.5,
+ "pos_y": 7.28125,
+ "pos_z": -144.96875,
+ "stations": "Berezin's Folly"
+ },
+ {
+ "name": "Hyades Sector MX-T c3-18",
+ "pos_x": 113.4375,
+ "pos_y": -22.625,
+ "pos_z": -119.03125,
+ "stations": "Martins Depot"
+ },
+ {
+ "name": "Hyades Sector MX-T c3-23",
+ "pos_x": 100.34375,
+ "pos_y": -1.96875,
+ "pos_z": -124.46875,
+ "stations": "Schweinfurth Terminal"
+ },
+ {
+ "name": "Hyades Sector MX-T c3-25",
+ "pos_x": 100.09375,
+ "pos_y": -10.84375,
+ "pos_z": -113.625,
+ "stations": "Crumey Vision,Silves Stop"
+ },
+ {
+ "name": "Hyades Sector MX-T c3-26",
+ "pos_x": 111.09375,
+ "pos_y": -21.5,
+ "pos_z": -132.40625,
+ "stations": "Alenquer Landing"
+ },
+ {
+ "name": "Hyades Sector MY-P b6-5",
+ "pos_x": 29.4375,
+ "pos_y": 36.0625,
+ "pos_z": -143.6875,
+ "stations": "Nicollier Depot,Panshin's Folly"
+ },
+ {
+ "name": "Hyades Sector NS-T c3-8",
+ "pos_x": 90.25,
+ "pos_y": -46.21875,
+ "pos_z": -135.1875,
+ "stations": "Gwynn Point,Herreshoff Enterprise"
+ },
+ {
+ "name": "Hyades Sector NU-N b7-4",
+ "pos_x": -117.15625,
+ "pos_y": 0.46875,
+ "pos_z": -108.875,
+ "stations": "Laird Installation"
+ },
+ {
+ "name": "Hyades Sector OI-S c4-22",
+ "pos_x": 100.15625,
+ "pos_y": 28.3125,
+ "pos_z": -104.1875,
+ "stations": "Coke Mine,Guerrero Enterprise"
+ },
+ {
+ "name": "Hyades Sector ON-T c3-14",
+ "pos_x": 22.96875,
+ "pos_y": -102,
+ "pos_z": -144.53125,
+ "stations": "Smith Vision"
+ },
+ {
+ "name": "Hyades Sector OO-E a13-1",
+ "pos_x": 37.9375,
+ "pos_y": -27.75,
+ "pos_z": -142.65625,
+ "stations": "Bunch Observatory"
+ },
+ {
+ "name": "Hyades Sector OO-P b6-4",
+ "pos_x": -5.5625,
+ "pos_y": 7.59375,
+ "pos_z": -130.40625,
+ "stations": "Coblentz Silo"
+ },
+ {
+ "name": "Hyades Sector OP-N b7-2",
+ "pos_x": -129.375,
+ "pos_y": -10.78125,
+ "pos_z": -120.625,
+ "stations": "Rasmussen Plant"
+ },
+ {
+ "name": "Hyades Sector PD-S c4-9",
+ "pos_x": 90.59375,
+ "pos_y": 6.59375,
+ "pos_z": -86.65625,
+ "stations": "Matthews Point,North Prospect"
+ },
+ {
+ "name": "Hyades Sector PI-T c3-12",
+ "pos_x": 3.0625,
+ "pos_y": -113.03125,
+ "pos_z": -124.6875,
+ "stations": "Hardy Vision"
+ },
+ {
+ "name": "Hyades Sector PI-T c3-14",
+ "pos_x": 4.65625,
+ "pos_y": -117.15625,
+ "pos_z": -118.5,
+ "stations": "Barbaro Installation,Guidoni Beacon"
+ },
+ {
+ "name": "Hyades Sector PJ-E a13-1",
+ "pos_x": 26.1875,
+ "pos_y": -43.375,
+ "pos_z": -143.5625,
+ "stations": "Arnason Arsenal"
+ },
+ {
+ "name": "Hyades Sector QE-O b7-3",
+ "pos_x": 17.90625,
+ "pos_y": 45.59375,
+ "pos_z": -122.125,
+ "stations": "Weinbaum Retreat"
+ },
+ {
+ "name": "Hyades Sector QN-T c3-17",
+ "pos_x": 131.34375,
+ "pos_y": -69.65625,
+ "pos_z": -136.40625,
+ "stations": "Cadamosto's Inheritance"
+ },
+ {
+ "name": "Hyades Sector QN-T c3-19",
+ "pos_x": 118.5625,
+ "pos_y": -85.71875,
+ "pos_z": -122.875,
+ "stations": "Fuca Vision"
+ },
+ {
+ "name": "Hyades Sector QN-T c3-21",
+ "pos_x": 102.125,
+ "pos_y": -97.125,
+ "pos_z": -138.4375,
+ "stations": "Matthews Keep"
+ },
+ {
+ "name": "Hyades Sector RE-O b7-4",
+ "pos_x": 52,
+ "pos_y": 50.90625,
+ "pos_z": -121.96875,
+ "stations": "Lem Beacon"
+ },
+ {
+ "name": "Hyades Sector RI-T c3-10",
+ "pos_x": 75.4375,
+ "pos_y": -142.375,
+ "pos_z": -125.78125,
+ "stations": "Carver Enterprise"
+ },
+ {
+ "name": "Hyades Sector RO-P b6-1",
+ "pos_x": 41.34375,
+ "pos_y": 12.78125,
+ "pos_z": -142.1875,
+ "stations": "Lundwall Beacon,Ricardo Base"
+ },
+ {
+ "name": "Hyades Sector RT-P b6-3",
+ "pos_x": 91.5,
+ "pos_y": 31.5625,
+ "pos_z": -133.375,
+ "stations": "Gooch Beacon"
+ },
+ {
+ "name": "Hyades Sector SJ-P b6-1",
+ "pos_x": 27.75,
+ "pos_y": -23.40625,
+ "pos_z": -143.25,
+ "stations": "He Vision"
+ },
+ {
+ "name": "Hyades Sector SO-E a13-2",
+ "pos_x": 75.0625,
+ "pos_y": -32.75,
+ "pos_z": -137.90625,
+ "stations": "Shiner Landing,Ryazanski Prospect"
+ },
+ {
+ "name": "Hyades Sector ST-Q b5-4",
+ "pos_x": 18.15625,
+ "pos_y": -48.875,
+ "pos_z": -164.125,
+ "stations": "Fernandez Relay"
+ },
+ {
+ "name": "Hyades Sector ST-Q b5-6",
+ "pos_x": 34,
+ "pos_y": -56.1875,
+ "pos_z": -154.53125,
+ "stations": "McCaffrey Installation"
+ },
+ {
+ "name": "Hyades Sector SY-R c4-16",
+ "pos_x": 124.375,
+ "pos_y": -52.1875,
+ "pos_z": -98.34375,
+ "stations": "Zettel Relay,Neville's Exile"
+ },
+ {
+ "name": "Hyades Sector TO-P b6-3",
+ "pos_x": 88.5,
+ "pos_y": 13.84375,
+ "pos_z": -138.3125,
+ "stations": "Schmidt Works,Cramer Reach"
+ },
+ {
+ "name": "Hyades Sector TT-Q b5-5",
+ "pos_x": 42.28125,
+ "pos_y": -45.625,
+ "pos_z": -157.8125,
+ "stations": "Gulyaev Bastion"
+ },
+ {
+ "name": "Hyades Sector TU-C a14-2",
+ "pos_x": 47.28125,
+ "pos_y": -34.34375,
+ "pos_z": -135,
+ "stations": "Poisson Depot"
+ },
+ {
+ "name": "Hyades Sector TU-N b7-4",
+ "pos_x": 7.1875,
+ "pos_y": -0.3125,
+ "pos_z": -120.84375,
+ "stations": "Busch Keep"
+ },
+ {
+ "name": "Hyades Sector UO-P b6-0",
+ "pos_x": 99.71875,
+ "pos_y": 0.5625,
+ "pos_z": -144.96875,
+ "stations": "Baker Landing"
+ },
+ {
+ "name": "Hyades Sector UO-R c4-9",
+ "pos_x": 29,
+ "pos_y": -124.8125,
+ "pos_z": -99.875,
+ "stations": "Abetti Settlement"
+ },
+ {
+ "name": "Hyades Sector UT-R c4-14",
+ "pos_x": 124.6875,
+ "pos_y": -97.625,
+ "pos_z": -88.53125,
+ "stations": "Sitterly Holdings"
+ },
+ {
+ "name": "Hyades Sector UT-R c4-22",
+ "pos_x": 121.1875,
+ "pos_y": -76.84375,
+ "pos_z": -99.5625,
+ "stations": "Samuda Arsenal"
+ },
+ {
+ "name": "Hyades Sector UV-L b8-5",
+ "pos_x": -101.65625,
+ "pos_y": -9.8125,
+ "pos_z": -96.21875,
+ "stations": "Marconi Installation,Henderson Prospect,White Enterprise"
+ },
+ {
+ "name": "Hyades Sector VJ-R c4-8",
+ "pos_x": -15.375,
+ "pos_y": -159.625,
+ "pos_z": -102.46875,
+ "stations": "Thompson Depot"
+ },
+ {
+ "name": "Hyades Sector VO-Q b5-3",
+ "pos_x": 45.25,
+ "pos_y": -71.125,
+ "pos_z": -150.6875,
+ "stations": "Crown Base,MacLean Enterprise"
+ },
+ {
+ "name": "Hyades Sector WJ-P b6-0",
+ "pos_x": 106.40625,
+ "pos_y": -7.625,
+ "pos_z": -139.9375,
+ "stations": "Arrhenius Enterprise"
+ },
+ {
+ "name": "Hyades Sector WJ-P b6-1",
+ "pos_x": 112.40625,
+ "pos_y": -22.8125,
+ "pos_z": -134.5,
+ "stations": "Plucker Landing,Bertin Installation"
+ },
+ {
+ "name": "Hyades Sector XB-K b9-5",
+ "pos_x": -107.15625,
+ "pos_y": -10.125,
+ "pos_z": -75.875,
+ "stations": "Lee Silo,Dampier Holdings"
+ },
+ {
+ "name": "Hyades Sector YZ-O b6-3",
+ "pos_x": 71,
+ "pos_y": -56.5625,
+ "pos_z": -136.09375,
+ "stations": "de Sousa Terminal"
+ },
+ {
+ "name": "Hyades Sector ZU-O b6-0",
+ "pos_x": 40.15625,
+ "pos_y": -83.5625,
+ "pos_z": -142.125,
+ "stations": "Pike's Inheritance"
+ },
+ {
+ "name": "Hydrae Sector AQ-Y c11",
+ "pos_x": 50.5,
+ "pos_y": 101.875,
+ "pos_z": 42.21875,
+ "stations": "Fanning Penal colony"
+ },
+ {
+ "name": "Hydrae Sector CL-X b1-4",
+ "pos_x": 101.625,
+ "pos_y": 117.25,
+ "pos_z": 41.71875,
+ "stations": "Samuda Beacon,Smith Enterprise"
+ },
+ {
+ "name": "Hydrae Sector CL-Y d81",
+ "pos_x": 81.5625,
+ "pos_y": 121,
+ "pos_z": 70.0625,
+ "stations": "Tarentum Prospect"
+ },
+ {
+ "name": "Hydrae Sector CL-Y d95",
+ "pos_x": 82.5,
+ "pos_y": 104.625,
+ "pos_z": 117.8125,
+ "stations": "Caselberg Terminal"
+ },
+ {
+ "name": "Hydrae Sector CL-Y d97",
+ "pos_x": 82.96875,
+ "pos_y": 96.84375,
+ "pos_z": 106.75,
+ "stations": "Gregory Palace"
+ },
+ {
+ "name": "Hydrae Sector CW-V b2-3",
+ "pos_x": 67.03125,
+ "pos_y": 140.9375,
+ "pos_z": 55.78125,
+ "stations": "Gentle's Folly"
+ },
+ {
+ "name": "Hydrae Sector DB-X c1-13",
+ "pos_x": 80.15625,
+ "pos_y": 140.9375,
+ "pos_z": 78.4375,
+ "stations": "Wylie Bastion,Frechet Terminal"
+ },
+ {
+ "name": "Hydrae Sector DL-Y c18",
+ "pos_x": 60.03125,
+ "pos_y": 79.75,
+ "pos_z": 18.1875,
+ "stations": "Wilcutt Landing,Spielberg Base"
+ },
+ {
+ "name": "Hydrae Sector DL-Y d96",
+ "pos_x": 108.875,
+ "pos_y": 61.46875,
+ "pos_z": 66.21875,
+ "stations": "Barreiros Barracks,Witt Vision,Tull Observatory"
+ },
+ {
+ "name": "Hydrae Sector EL-Y c21",
+ "pos_x": 111.84375,
+ "pos_y": 86.0625,
+ "pos_z": 52.71875,
+ "stations": "Longyear Plant"
+ },
+ {
+ "name": "Hydrae Sector GW-W c1-12",
+ "pos_x": 128.5,
+ "pos_y": 97.28125,
+ "pos_z": 56.1875,
+ "stations": "Fu Landing"
+ },
+ {
+ "name": "Hydrae Sector GW-W c1-19",
+ "pos_x": 126.84375,
+ "pos_y": 114.71875,
+ "pos_z": 65.875,
+ "stations": "Gallun Terminal"
+ },
+ {
+ "name": "Hydrae Sector IR-W c1-11",
+ "pos_x": 120.90625,
+ "pos_y": 83.875,
+ "pos_z": 85.3125,
+ "stations": "Morgan Prospect"
+ },
+ {
+ "name": "Hydrae Sector IR-W c1-19",
+ "pos_x": 125.15625,
+ "pos_y": 86.1875,
+ "pos_z": 94.65625,
+ "stations": "Brendan Survey,Crumey Holdings"
+ },
+ {
+ "name": "Hydrae Sector IR-W c1-20",
+ "pos_x": 124.59375,
+ "pos_y": 82.28125,
+ "pos_z": 89.4375,
+ "stations": "Yu Plant"
+ },
+ {
+ "name": "Hydrae Sector JC-V c2-11",
+ "pos_x": 59.28125,
+ "pos_y": 99.1875,
+ "pos_z": 116,
+ "stations": "Herbert Terminal"
+ },
+ {
+ "name": "Hydrae Sector JC-V c2-5",
+ "pos_x": 74.90625,
+ "pos_y": 103.71875,
+ "pos_z": 99.8125,
+ "stations": "Pordenone Beacon"
+ },
+ {
+ "name": "Hydrae Sector JH-V b2-3",
+ "pos_x": 91.625,
+ "pos_y": 83.25,
+ "pos_z": 72.59375,
+ "stations": "Rice Installation"
+ },
+ {
+ "name": "Hydrae Sector NS-U c2-9",
+ "pos_x": 65.75,
+ "pos_y": 48.09375,
+ "pos_z": 102.3125,
+ "stations": "Thornycroft Point,Ziemkiewicz Terminal"
+ },
+ {
+ "name": "Hydrae Sector ON-T b3-1",
+ "pos_x": 99.875,
+ "pos_y": 92.96875,
+ "pos_z": 78.0625,
+ "stations": "Detention Vessel Epsilon"
+ },
+ {
+ "name": "Hydrae Sector QI-T b3-2",
+ "pos_x": 96.84375,
+ "pos_y": 74.5625,
+ "pos_z": 78.5625,
+ "stations": "Humphreys Settlement"
+ },
+ {
+ "name": "Hydrae Sector RT-R b4-2",
+ "pos_x": 81.15625,
+ "pos_y": 75.5,
+ "pos_z": 114.71875,
+ "stations": "Crown Refinery,Shiner Terminal"
+ },
+ {
+ "name": "Hydrae Sector ST-R b4-1",
+ "pos_x": 97.59375,
+ "pos_y": 83.40625,
+ "pos_z": 113.15625,
+ "stations": "Kingsmill Terminal,Webb Plant,Henslow Survey"
+ },
+ {
+ "name": "Hydrae Sector TD-T b3-1",
+ "pos_x": 119.3125,
+ "pos_y": 42.5625,
+ "pos_z": 75.53125,
+ "stations": "Bulleid Holdings,Tanaka Base"
+ },
+ {
+ "name": "Hydrae Sector ZE-A d80",
+ "pos_x": 111.1875,
+ "pos_y": 96.375,
+ "pos_z": 35.1875,
+ "stations": "Al-Kashi Enterprise,Wescott Point"
+ },
+ {
+ "name": "Hyel Ye",
+ "pos_x": 122,
+ "pos_y": 12.375,
+ "pos_z": 45.75,
+ "stations": "Read Settlement"
+ },
+ {
+ "name": "Hyel Yeh",
+ "pos_x": -96.28125,
+ "pos_y": 0.625,
+ "pos_z": -86.28125,
+ "stations": "Peary Enterprise,Hoshi City,Williams Enterprise,Carlisle's Folly,Bujold Terminal,Beekman Depot,Tayler Station,Minkowski Terminal,Malcolm Orbital"
+ },
+ {
+ "name": "Hyelobo Di",
+ "pos_x": 86.46875,
+ "pos_y": -131.1875,
+ "pos_z": 148.28125,
+ "stations": "Ryan Colony,Langley Dock"
+ },
+ {
+ "name": "Hyldekagati",
+ "pos_x": 19.25,
+ "pos_y": -64.53125,
+ "pos_z": -1.5625,
+ "stations": "Ingstad Terminal,Brunel Station,Sacco Orbital,Chaudhary Silo,Bamford Camp"
+ },
+ {
+ "name": "Hyldeptu",
+ "pos_x": 38.65625,
+ "pos_y": -9.28125,
+ "pos_z": -116.40625,
+ "stations": "Schroeder Gateway,Friesner Station,Narvaez Silo"
+ },
+ {
+ "name": "Hyperborea",
+ "pos_x": 45.59375,
+ "pos_y": -139.15625,
+ "pos_z": 22.4375,
+ "stations": "Dickinson Observatory,Casa Lofthus,Bushkov Dock"
+ },
+ {
+ "name": "Hyperion",
+ "pos_x": -51.1875,
+ "pos_y": 7.5,
+ "pos_z": -16.71875,
+ "stations": "Howe Platform,Rukavishnikov Installation"
+ },
+ {
+ "name": "Hyrokkin",
+ "pos_x": -29.6875,
+ "pos_y": -125.9375,
+ "pos_z": 25.28125,
+ "stations": "Brule Dock"
+ },
+ {
+ "name": "Hyroks",
+ "pos_x": -28.5625,
+ "pos_y": -2.09375,
+ "pos_z": 8.5,
+ "stations": "Watson Horizons,Krusvar Installation"
+ },
+ {
+ "name": "Hyroku",
+ "pos_x": 95.15625,
+ "pos_y": -2.9375,
+ "pos_z": -72.625,
+ "stations": "Rashid Platform"
+ },
+ {
+ "name": "i Bootis",
+ "pos_x": -22.375,
+ "pos_y": 34.84375,
+ "pos_z": 4,
+ "stations": "Maher Stellar Research,Chango Dock"
+ },
+ {
+ "name": "I Carinae",
+ "pos_x": 47.0625,
+ "pos_y": -13,
+ "pos_z": 20.3125,
+ "stations": "Somerset Station,Eddington Base,Lousma Keep,Vasyutin Landing,Culpeper Dock,Bokeili Prospect"
+ },
+ {
+ "name": "I Puppis",
+ "pos_x": 65.71875,
+ "pos_y": -19.09375,
+ "pos_z": -14.09375,
+ "stations": "Levinson City"
+ },
+ {
+ "name": "Iado",
+ "pos_x": -3.03125,
+ "pos_y": -158.125,
+ "pos_z": 55.875,
+ "stations": "Boscovich Terminal"
+ },
+ {
+ "name": "Iadoitj",
+ "pos_x": 76.03125,
+ "pos_y": -114.875,
+ "pos_z": 171.5625,
+ "stations": "Morrill Mine"
+ },
+ {
+ "name": "Iadou",
+ "pos_x": 116.625,
+ "pos_y": -120.1875,
+ "pos_z": -84.21875,
+ "stations": "Gustafsson Arena"
+ },
+ {
+ "name": "Iah",
+ "pos_x": 72.3125,
+ "pos_y": -26.3125,
+ "pos_z": -99,
+ "stations": "Sagan Vision"
+ },
+ {
+ "name": "Iah Bei",
+ "pos_x": 20.5625,
+ "pos_y": -120.1875,
+ "pos_z": -143.5625,
+ "stations": "Scortia Colony"
+ },
+ {
+ "name": "Iah Bulu",
+ "pos_x": -94.09375,
+ "pos_y": 39.84375,
+ "pos_z": 31.28125,
+ "stations": "Savery Hub,Metcalf Ring,Poindexter Hub,Westerfeld Gateway"
+ },
+ {
+ "name": "Iah Lanitei",
+ "pos_x": 62.15625,
+ "pos_y": -71.34375,
+ "pos_z": 13.40625,
+ "stations": "Dassault Gateway,Levinson Enterprise,Niijima Gateway"
+ },
+ {
+ "name": "Iah Lohra",
+ "pos_x": -41.6875,
+ "pos_y": -147.40625,
+ "pos_z": 27.34375,
+ "stations": "Fedden Orbital,Kalam Keep"
+ },
+ {
+ "name": "Iama Kheph",
+ "pos_x": 111.28125,
+ "pos_y": -168.71875,
+ "pos_z": 79.78125,
+ "stations": "Gustafsson's Folly,Skjellerup Port,Schumacher Beacon,Shoujing Platform,Qushji Terminal,Haarsma Barracks"
+ },
+ {
+ "name": "Iapo Vuh",
+ "pos_x": -114.46875,
+ "pos_y": -5.78125,
+ "pos_z": -43.4375,
+ "stations": "Barentsz Orbital,Fuchs Station,Penn Orbital,Ostrander Reach,Resnik Exchange,Bridger Terminal,Navigator Horizons"
+ },
+ {
+ "name": "Iapodes",
+ "pos_x": -97.125,
+ "pos_y": -11.78125,
+ "pos_z": -171.28125,
+ "stations": "Tsunenaga Dock,Salgari Beacon"
+ },
+ {
+ "name": "Iapori",
+ "pos_x": 99.90625,
+ "pos_y": 62.71875,
+ "pos_z": 0.0625,
+ "stations": "al-Din Hub,Volkov Port,Hadfield Station"
+ },
+ {
+ "name": "Ibibara",
+ "pos_x": 57.375,
+ "pos_y": -20.03125,
+ "pos_z": 100.125,
+ "stations": "Phillips City,Davis Hub,Flammarion Terminal"
+ },
+ {
+ "name": "Ibibe",
+ "pos_x": -11.0625,
+ "pos_y": 21.625,
+ "pos_z": 142.4375,
+ "stations": "Hurley Plant"
+ },
+ {
+ "name": "Ibibio",
+ "pos_x": -32.4375,
+ "pos_y": -184.09375,
+ "pos_z": 36.78125,
+ "stations": "Lilienthal Landing,Robinson Landing"
+ },
+ {
+ "name": "Ibisanyan",
+ "pos_x": 150.65625,
+ "pos_y": -236.4375,
+ "pos_z": -5.28125,
+ "stations": "Kuo Mines,Brosnatch Terminal,Humason Prospect,Emshwiller Terminal"
+ },
+ {
+ "name": "Ibishia",
+ "pos_x": -6.6875,
+ "pos_y": 33.875,
+ "pos_z": -116.1875,
+ "stations": "Montrose Lab,Fawcett Colony,Wood Camp"
+ },
+ {
+ "name": "Ibor",
+ "pos_x": 61.375,
+ "pos_y": -59.71875,
+ "pos_z": -73.28125,
+ "stations": "Simpson Port,Shargin Settlement"
+ },
+ {
+ "name": "Iboraga",
+ "pos_x": -30.28125,
+ "pos_y": -151.40625,
+ "pos_z": 0.5625,
+ "stations": "Abe Ring,Wilhelm Klinkerfues Colony,Naboth Gateway,Hooker Observatory"
+ },
+ {
+ "name": "Ibormbu",
+ "pos_x": 3.46875,
+ "pos_y": -154.0625,
+ "pos_z": -3.8125,
+ "stations": "Gunter Hub,Freas Survey,Addams Hub"
+ },
+ {
+ "name": "Iburoana",
+ "pos_x": -18.15625,
+ "pos_y": 134.40625,
+ "pos_z": 4.375,
+ "stations": "Darlton Colony"
+ },
+ {
+ "name": "Iburodo",
+ "pos_x": 70.40625,
+ "pos_y": 79.28125,
+ "pos_z": 92.6875,
+ "stations": "Archimedes Colony,Harper Relay"
+ },
+ {
+ "name": "Iburongin",
+ "pos_x": -181.0625,
+ "pos_y": 70.53125,
+ "pos_z": 85.28125,
+ "stations": "Womack Outpost"
+ },
+ {
+ "name": "Iburri",
+ "pos_x": 129.625,
+ "pos_y": -166.5,
+ "pos_z": 150.6875,
+ "stations": "Paczynski Mine,Tully Relay,Raleigh Hub"
+ },
+ {
+ "name": "IC 1805 Sector AV-O c6-6",
+ "pos_x": -4336.9375,
+ "pos_y": 12.84375,
+ "pos_z": -4423.15625,
+ "stations": "Sagan Class Tourist Ship"
+ },
+ {
+ "name": "Iceni",
+ "pos_x": 62.9375,
+ "pos_y": 1.8125,
+ "pos_z": 141.375,
+ "stations": "Grandin Dock,Soper Lab"
+ },
+ {
+ "name": "Iceniguari",
+ "pos_x": -152.84375,
+ "pos_y": -42.53125,
+ "pos_z": -39.1875,
+ "stations": "Beatty Settlement"
+ },
+ {
+ "name": "Icones",
+ "pos_x": 163.6875,
+ "pos_y": -40.625,
+ "pos_z": -33.03125,
+ "stations": "Leavitt Base"
+ },
+ {
+ "name": "Iconti Shi",
+ "pos_x": 115.71875,
+ "pos_y": -243.96875,
+ "pos_z": 32.96875,
+ "stations": "Jones Gateway,Dyson Station,Brorsen Port,Hiraga Silo"
+ },
+ {
+ "name": "Icontia",
+ "pos_x": -64.8125,
+ "pos_y": 24.875,
+ "pos_z": 19.3125,
+ "stations": "Betancourt Refinery"
+ },
+ {
+ "name": "Icovit",
+ "pos_x": -22.75,
+ "pos_y": 15.75,
+ "pos_z": 97.96875,
+ "stations": "McDivitt Installation,Euler Holdings"
+ },
+ {
+ "name": "Ictino",
+ "pos_x": 113.5625,
+ "pos_y": -12.8125,
+ "pos_z": 22.4375,
+ "stations": "Gantt Refinery,Pirsan Silo,Messerschmid Port"
+ },
+ {
+ "name": "ICZ KS-T b3-5",
+ "pos_x": -2.84375,
+ "pos_y": -143.3125,
+ "pos_z": 42.75,
+ "stations": "The Fist of the Empire"
+ },
+ {
+ "name": "ICZ ZK-X b1-0",
+ "pos_x": -10.96875,
+ "pos_y": -121.5625,
+ "pos_z": 11.4375,
+ "stations": "The Seraph's Wing"
+ },
+ {
+ "name": "Ida Dhor",
+ "pos_x": 128.34375,
+ "pos_y": 6.46875,
+ "pos_z": 16.78125,
+ "stations": "Vancouver Gateway,Thompson Enterprise,Patterson Orbital"
+ },
+ {
+ "name": "Idin",
+ "pos_x": 76.9375,
+ "pos_y": -163.40625,
+ "pos_z": -3.15625,
+ "stations": "Secchi Station,Wilhelm Klinkerfues' Progress"
+ },
+ {
+ "name": "Idinda",
+ "pos_x": -86.8125,
+ "pos_y": -64.59375,
+ "pos_z": -21.15625,
+ "stations": "Poindexter Hub,Kepler Port,Bean Port,Bernard Gateway,Savery Port,O'Connor Gateway,Sleator Terminal,Petaja Depot,Fullerton Terminal,Dann Settlement"
+ },
+ {
+ "name": "Idini",
+ "pos_x": -80,
+ "pos_y": 30.59375,
+ "pos_z": -118.65625,
+ "stations": "Gurney Enterprise,Garfinkle Depot,Ochoa Vision"
+ },
+ {
+ "name": "Idoma",
+ "pos_x": -75.34375,
+ "pos_y": -21.6875,
+ "pos_z": -100.90625,
+ "stations": "Lebesgue Stop"
+ },
+ {
+ "name": "Idomaguara",
+ "pos_x": 140.5,
+ "pos_y": -142.0625,
+ "pos_z": 117.6875,
+ "stations": "Royo Stop"
+ },
+ {
+ "name": "Idoman",
+ "pos_x": 77.875,
+ "pos_y": -40.78125,
+ "pos_z": -119.125,
+ "stations": "Bao Silo,Clement Outpost"
+ },
+ {
+ "name": "Idomatyritu",
+ "pos_x": 145.09375,
+ "pos_y": -69.09375,
+ "pos_z": -12.375,
+ "stations": "Garrido Holdings,Shaw Station,Gresh Depot,Frimout Dock,Stjepan Seljan Enterprise"
+ },
+ {
+ "name": "Idu mishmi",
+ "pos_x": 59.84375,
+ "pos_y": -198.6875,
+ "pos_z": 62.96875,
+ "stations": "Arnold Base"
+ },
+ {
+ "name": "Idu misi",
+ "pos_x": -56.875,
+ "pos_y": 29.15625,
+ "pos_z": 102.78125,
+ "stations": "Collins Point,Otiman Terminal,Cseszneky Ring,Nikitin Vision"
+ },
+ {
+ "name": "Idunei",
+ "pos_x": 155.25,
+ "pos_y": -186.59375,
+ "pos_z": 5.46875,
+ "stations": "Dickinson Platform,Artsutanov Dock,Bohrmann Dock,Navigator Holdings"
+ },
+ {
+ "name": "Idung Yin",
+ "pos_x": 31.71875,
+ "pos_y": -142.375,
+ "pos_z": 7.1875,
+ "stations": "Husband Horizons,Greenstein Orbital,Fleming Platform"
+ },
+ {
+ "name": "Idunga",
+ "pos_x": -22.8125,
+ "pos_y": 135,
+ "pos_z": -44.71875,
+ "stations": "Thompson Colony"
+ },
+ {
+ "name": "Idungbates",
+ "pos_x": 42.8125,
+ "pos_y": -37.875,
+ "pos_z": 87.5,
+ "stations": "Pryor Dock"
+ },
+ {
+ "name": "Iduni",
+ "pos_x": -139.375,
+ "pos_y": -80.125,
+ "pos_z": -18.4375,
+ "stations": "Tucker Lab,Savorgnan de Brazza Survey"
+ },
+ {
+ "name": "Idunn",
+ "pos_x": -14.125,
+ "pos_y": -170.03125,
+ "pos_z": 91.59375,
+ "stations": "Kandrup Port,Young City,Corben Station,Amnuel's Claim"
+ },
+ {
+ "name": "Idununn",
+ "pos_x": -84.09375,
+ "pos_y": 20.4375,
+ "pos_z": 39.71875,
+ "stations": "Steiner Dock,Cayley Dock,Nansen Lab,Barnaby Terminal,Bao Gateway,Mallett Terminal,Quinn Gateway,Frobisher City,Banks Port,Lewitt Enterprise,Hedley Exchange"
+ },
+ {
+ "name": "Idzumoku",
+ "pos_x": -50.8125,
+ "pos_y": 63.59375,
+ "pos_z": 41.1875,
+ "stations": "Ito Prospect,Barnes Terminal"
+ },
+ {
+ "name": "Ienpalang",
+ "pos_x": 133.53125,
+ "pos_y": -99.75,
+ "pos_z": 52.65625,
+ "stations": "Bradley Gateway,Schuster Station,Brundage City,Kidd Observatory,Bordage Settlement,Gunn Beacon,Chertovsky Gateway,Doyle Survey"
+ },
+ {
+ "name": "Igae",
+ "pos_x": 34.5625,
+ "pos_y": -6.90625,
+ "pos_z": 96.96875,
+ "stations": "Jahn Point,Lynn Prospect,Kubasov Terminal"
+ },
+ {
+ "name": "Igaenisan",
+ "pos_x": -58.25,
+ "pos_y": -76.9375,
+ "pos_z": -11.59375,
+ "stations": "Liebig Ring,Knapp Dock,Garn Vision,Altshuller Horizons,Zalyotin Hub"
+ },
+ {
+ "name": "Igal",
+ "pos_x": 79.375,
+ "pos_y": -175.96875,
+ "pos_z": -0.46875,
+ "stations": "Shapley Dock,Tapinas Depot,Qushji Orbital,Hirayama Terminal,Vuia Station,Firsoff Orbital,Helin Port,Bouvard Hub,Abbott Horizons,Vlaicu Station"
+ },
+ {
+ "name": "Igala",
+ "pos_x": -89.84375,
+ "pos_y": 23.0625,
+ "pos_z": -24.375,
+ "stations": "Noakes Hub,Agnesi City,Feoktistov Terminal,Fiennes Landing"
+ },
+ {
+ "name": "Igalalani",
+ "pos_x": -16.34375,
+ "pos_y": -33.625,
+ "pos_z": 104.4375,
+ "stations": "Chorel Hub"
+ },
+ {
+ "name": "Igaledonii",
+ "pos_x": 118.21875,
+ "pos_y": -30.84375,
+ "pos_z": 67.375,
+ "stations": "Hausdorff Beacon,Tem Outpost"
+ },
+ {
+ "name": "Igali",
+ "pos_x": -2.75,
+ "pos_y": 38.875,
+ "pos_z": -144.25,
+ "stations": "Moore Prospect,Klink Camp,Wollheim Settlement"
+ },
+ {
+ "name": "Igalilinima",
+ "pos_x": -72.59375,
+ "pos_y": 15.84375,
+ "pos_z": 86.6875,
+ "stations": "Carey Enterprise,Maxwell Dock,Armstrong Enterprise,Mattingly Orbital,Cogswell Settlement,Mondeh Gateway,Hauck Ring,Tasman Legacy"
+ },
+ {
+ "name": "Igaluk",
+ "pos_x": 3.96875,
+ "pos_y": 129.90625,
+ "pos_z": -7.25,
+ "stations": "Jensen Orbital"
+ },
+ {
+ "name": "Igalumathi",
+ "pos_x": 81.21875,
+ "pos_y": 11.90625,
+ "pos_z": 121.84375,
+ "stations": "Eyharts Hub,Bridges Terminal,Szilard Orbital,Katzenstein's Folly"
+ },
+ {
+ "name": "Igbirikan",
+ "pos_x": -54.6875,
+ "pos_y": 12.59375,
+ "pos_z": 103.40625,
+ "stations": "Lyakhov Station,Bacigalupi Enterprise,Barratt Hub,Onizuka Penal colony"
+ },
+ {
+ "name": "Igbo Di",
+ "pos_x": -99.03125,
+ "pos_y": 78.875,
+ "pos_z": 78.40625,
+ "stations": "Bluford Depot,Bartoe Terminal,Laplace Settlement"
+ },
+ {
+ "name": "Igbo Kora",
+ "pos_x": 139.8125,
+ "pos_y": -82.4375,
+ "pos_z": -69.34375,
+ "stations": "Palitzsch City,Shunkai Plant"
+ },
+ {
+ "name": "Igbon",
+ "pos_x": -48.28125,
+ "pos_y": -31.03125,
+ "pos_z": -112.5625,
+ "stations": "Eilenberg Landing"
+ },
+ {
+ "name": "Igbonii",
+ "pos_x": 35.125,
+ "pos_y": 155.71875,
+ "pos_z": 87.1875,
+ "stations": "Janifer Point,Wellman Depot,Knapp Outpost,Lasswitz Vista"
+ },
+ {
+ "name": "Igboniu",
+ "pos_x": 128.53125,
+ "pos_y": 71.25,
+ "pos_z": 26.78125,
+ "stations": "Harness City,Nordenskiold Enterprise,Alexander City,Bates Base"
+ },
+ {
+ "name": "Igores",
+ "pos_x": 116.625,
+ "pos_y": -95.875,
+ "pos_z": 87.46875,
+ "stations": "Parker Beacon,Bouvard Port,Plante Bastion"
+ },
+ {
+ "name": "Igoria",
+ "pos_x": 118.75,
+ "pos_y": -198.59375,
+ "pos_z": 19.875,
+ "stations": "Jones Base,Khrenov Bastion,Dowty Works"
+ },
+ {
+ "name": "Igorisa",
+ "pos_x": 144.8125,
+ "pos_y": -10.03125,
+ "pos_z": -20.625,
+ "stations": "Roberts Platform,Silva Installation"
+ },
+ {
+ "name": "Igorodimos",
+ "pos_x": -13.78125,
+ "pos_y": -20.4375,
+ "pos_z": 96.21875,
+ "stations": "Delbruck Ring"
+ },
+ {
+ "name": "Igorot",
+ "pos_x": -172.15625,
+ "pos_y": 0.84375,
+ "pos_z": -43.75,
+ "stations": "Humboldt Colony,Gagnan Port"
+ },
+ {
+ "name": "Ijo",
+ "pos_x": 74.4375,
+ "pos_y": 63.6875,
+ "pos_z": -106.09375,
+ "stations": "Kanwar Mines,Piper Camp,Bentham's Inheritance"
+ },
+ {
+ "name": "Ikenga",
+ "pos_x": -19.8125,
+ "pos_y": -35.875,
+ "pos_z": -46,
+ "stations": "Yegorov Orbital,Sellers Port,Lorenz Station,Kornbluth Depot,Pauli Gateway,Balandin's Progress,Leoniceno Station"
+ },
+ {
+ "name": "Ikpe",
+ "pos_x": 44.625,
+ "pos_y": -190.09375,
+ "pos_z": 84.4375,
+ "stations": "Wolf Relay,Haro Point"
+ },
+ {
+ "name": "Ikpen",
+ "pos_x": -105.6875,
+ "pos_y": 63.96875,
+ "pos_z": -72.1875,
+ "stations": "Wankel Legacy"
+ },
+ {
+ "name": "Ikpenones",
+ "pos_x": -39.375,
+ "pos_y": 31.5625,
+ "pos_z": -45.09375,
+ "stations": "Fuchs Silo,Salam Terminal,Still Terminal,Stasheff Laboratory,Chaudhary Orbital"
+ },
+ {
+ "name": "Iksvaku",
+ "pos_x": -162.4375,
+ "pos_y": -38.03125,
+ "pos_z": 0.09375,
+ "stations": "Zamka Mines,Murdoch Platform,Hahn Prospect"
+ },
+ {
+ "name": "Iksvatan",
+ "pos_x": -12.6875,
+ "pos_y": -60.125,
+ "pos_z": 116.46875,
+ "stations": "Maxwell Port,Fuchs Arsenal,Grover Port,Bean Port"
+ },
+ {
+ "name": "Iksvati",
+ "pos_x": -58.59375,
+ "pos_y": -2.0625,
+ "pos_z": 126.40625,
+ "stations": "Olsen Horizons,Hoffman Outpost"
+ },
+ {
+ "name": "Iktock",
+ "pos_x": 112.03125,
+ "pos_y": -144.28125,
+ "pos_z": 66.375,
+ "stations": "Folland Ring,Pimi Colony,Beaumont Camp,McKee Vision,Wegener Depot"
+ },
+ {
+ "name": "Iktomisi",
+ "pos_x": -137.5,
+ "pos_y": -68.40625,
+ "pos_z": 2.375,
+ "stations": "Behnken Station"
+ },
+ {
+ "name": "IL Aquarii",
+ "pos_x": -6.15625,
+ "pos_y": -13.34375,
+ "pos_z": 4.78125,
+ "stations": "Kinsey Enterprise,Gagnan Terminal,Patsayev Station"
+ },
+ {
+ "name": "Ilbandi",
+ "pos_x": 113.625,
+ "pos_y": 67.21875,
+ "pos_z": 2.625,
+ "stations": "Lee Escape,Crown Survey"
+ },
+ {
+ "name": "Ilbassi",
+ "pos_x": 87.5625,
+ "pos_y": 61.0625,
+ "pos_z": 102.28125,
+ "stations": "Virtanen Works,Wang Landing"
+ },
+ {
+ "name": "Ildaker",
+ "pos_x": -125.90625,
+ "pos_y": -2.90625,
+ "pos_z": -8.8125,
+ "stations": "Kronecker Platform"
+ },
+ {
+ "name": "Ildana",
+ "pos_x": 33.90625,
+ "pos_y": -18,
+ "pos_z": 141.125,
+ "stations": "Griggs Works,Bose Port,Diamond Survey"
+ },
+ {
+ "name": "Ildano",
+ "pos_x": 98.96875,
+ "pos_y": -58.6875,
+ "pos_z": -131.71875,
+ "stations": "Piaget Beacon,Massimino Sanctuary"
+ },
+ {
+ "name": "Ilmeno",
+ "pos_x": -20.6875,
+ "pos_y": -217.90625,
+ "pos_z": -41.96875,
+ "stations": "Paczynski Relay,Kojima Vision,Veblen Works"
+ },
+ {
+ "name": "Ilmenses",
+ "pos_x": 4.21875,
+ "pos_y": 12.5,
+ "pos_z": 168.46875,
+ "stations": "Barry Works,Strekalov Landing"
+ },
+ {
+ "name": "Ilmesci",
+ "pos_x": -16.59375,
+ "pos_y": -140.8125,
+ "pos_z": 56.90625,
+ "stations": "Argelander Mines,Nixon Prospect,Melotte Vision"
+ },
+ {
+ "name": "Ilya Nigh",
+ "pos_x": 82.28125,
+ "pos_y": -118.125,
+ "pos_z": -35.78125,
+ "stations": "Thomson City,Lyot Vision"
+ },
+ {
+ "name": "Ilyaqa",
+ "pos_x": 97.8125,
+ "pos_y": -100.59375,
+ "pos_z": -46.96875,
+ "stations": "Stebbins Hub"
+ },
+ {
+ "name": "Iman Caber",
+ "pos_x": 16.09375,
+ "pos_y": -19.90625,
+ "pos_z": 24.46875,
+ "stations": "Dezhurov Enterprise,Payette Hub,Dobrovolski Station,Magnus Orbital,Guest Station,Savery Terminal,Cook Survey,Cantor Holdings,Barth Point,Lamarck Ring,Ashby Installation,Hart Point,Vittori Port"
+ },
+ {
+ "name": "Imango",
+ "pos_x": -50.59375,
+ "pos_y": -2.03125,
+ "pos_z": -93.5625,
+ "stations": "Heisenberg City"
+ },
+ {
+ "name": "Imani",
+ "pos_x": -38.625,
+ "pos_y": -77.125,
+ "pos_z": -41.5625,
+ "stations": "Ewald Survey,Lindsey Settlement,Meucci Horizons,Andersson Holdings"
+ },
+ {
+ "name": "Imantana",
+ "pos_x": -42.625,
+ "pos_y": -85.375,
+ "pos_z": -34.25,
+ "stations": "Brongniart Orbital,Grover Terminal,Melvill Port,Cassidy Refinery,Back Prospect"
+ },
+ {
+ "name": "Imen",
+ "pos_x": -20.75,
+ "pos_y": 15.40625,
+ "pos_z": 132.25,
+ "stations": "Crown Survey,Gelfand Relay"
+ },
+ {
+ "name": "Imen Mina",
+ "pos_x": -80.34375,
+ "pos_y": -17.40625,
+ "pos_z": 113.75,
+ "stations": "Savitskaya Enterprise,Neumann Station,Oswald Colony"
+ },
+ {
+ "name": "Imenhit",
+ "pos_x": -40.375,
+ "pos_y": -17,
+ "pos_z": 82.8125,
+ "stations": "Young Settlement,Jones Relay,Clement City,Mayer Beacon,Ryman Hub"
+ },
+ {
+ "name": "Imentet",
+ "pos_x": 45.9375,
+ "pos_y": -2.625,
+ "pos_z": -122,
+ "stations": "Chamitoff Outpost,Isherwood Holdings,Meucci Mines"
+ },
+ {
+ "name": "Imeut",
+ "pos_x": 89.03125,
+ "pos_y": -72.25,
+ "pos_z": -103.0625,
+ "stations": "Ampere Dock,Levi-Strauss Hub,Wilkes Base"
+ },
+ {
+ "name": "Imeutrimudj",
+ "pos_x": -56.375,
+ "pos_y": -67.65625,
+ "pos_z": -21.625,
+ "stations": "Goddard Dock,Robinson Landing,Korniyenko Terminal,Deb Orbital,Stebler Terminal,Levy Gateway,Hirase Port,Prunariu Station,Lessing Vista"
+ },
+ {
+ "name": "Imeuts",
+ "pos_x": 39.28125,
+ "pos_y": 5.75,
+ "pos_z": 56.25,
+ "stations": "Pippin Installation,Clark Terminal"
+ },
+ {
+ "name": "Imeutsu",
+ "pos_x": -59.09375,
+ "pos_y": -159.875,
+ "pos_z": -41.75,
+ "stations": "Frick Enterprise,Ashby Hub,Braun Orbital,Cartan Camp,Macan Relay"
+ },
+ {
+ "name": "Imhote",
+ "pos_x": -16.96875,
+ "pos_y": -75.4375,
+ "pos_z": 86.625,
+ "stations": "Fabian Orbital"
+ },
+ {
+ "name": "Imhoth",
+ "pos_x": -45.46875,
+ "pos_y": 59.875,
+ "pos_z": -61.875,
+ "stations": "Baraniecki Hub"
+ },
+ {
+ "name": "Imhotian",
+ "pos_x": -44.21875,
+ "pos_y": 5.59375,
+ "pos_z": 110.71875,
+ "stations": "Grunsfeld Port,Langford Installation"
+ },
+ {
+ "name": "Imhottii",
+ "pos_x": -16,
+ "pos_y": -114,
+ "pos_z": 123.8125,
+ "stations": "la Cosa Oasis,Woodroffe Platform,Folland Port"
+ },
+ {
+ "name": "Imisses",
+ "pos_x": -68.125,
+ "pos_y": 38.96875,
+ "pos_z": 39.875,
+ "stations": "Shkaplerov Works,Jemison Settlement,Morgan's Inheritance"
+ },
+ {
+ "name": "Imiutli",
+ "pos_x": -84.0625,
+ "pos_y": -43.71875,
+ "pos_z": -13.5,
+ "stations": "Harris Station"
+ },
+ {
+ "name": "Imset",
+ "pos_x": 158.65625,
+ "pos_y": -8.28125,
+ "pos_z": 30.1875,
+ "stations": "Barentsz Dock,Walters City,Czerny Terminal,Stross City,von Biela Base"
+ },
+ {
+ "name": "Imsetani",
+ "pos_x": 52.5,
+ "pos_y": 39.0625,
+ "pos_z": -82.9375,
+ "stations": "Vizcaino Port,Bridger Observatory,Quick Survey"
+ },
+ {
+ "name": "Inara",
+ "pos_x": -93.8125,
+ "pos_y": 39.71875,
+ "pos_z": -86.15625,
+ "stations": "Citi Gateway,Hardy Depot,Krupkat Dock,Bombelli Hub,Gordon Base,Piercy Stop,Koontz Port,Willis Dock,Moore Terminal,Paulo da Gama Hub,Normand City,Bachman Observatory,Christopher Station,Sladek Settlement,Stefansson Holdings"
+ },
+ {
+ "name": "Inarajajara",
+ "pos_x": -81.21875,
+ "pos_y": -93.96875,
+ "pos_z": -38.8125,
+ "stations": "Laval Terminal,Kuhn Landing,Volkov Enterprise,Bailey Landing"
+ },
+ {
+ "name": "Inars",
+ "pos_x": 162.9375,
+ "pos_y": -152.625,
+ "pos_z": 115.96875,
+ "stations": "Chawla Dock,Green Hub,Kirby Terminal"
+ },
+ {
+ "name": "Inawyddi",
+ "pos_x": 85.875,
+ "pos_y": -102.8125,
+ "pos_z": -135.40625,
+ "stations": "Bierce Beacon"
+ },
+ {
+ "name": "Indaol",
+ "pos_x": -14.03125,
+ "pos_y": 79.21875,
+ "pos_z": -3.59375,
+ "stations": "Archer Settlement,Geronimo,Wafer Landing,Vries Vision"
+ },
+ {
+ "name": "Indi",
+ "pos_x": 50.84375,
+ "pos_y": 127.40625,
+ "pos_z": 108.25,
+ "stations": "Crook Works,Rand Survey,Arnarson's Progress"
+ },
+ {
+ "name": "Indians",
+ "pos_x": -22.84375,
+ "pos_y": 156.53125,
+ "pos_z": -19.75,
+ "stations": "Friesner Base,Vaez de Torres' Inheritance"
+ },
+ {
+ "name": "Indines",
+ "pos_x": 28.21875,
+ "pos_y": 173.15625,
+ "pos_z": -31.15625,
+ "stations": "England Point"
+ },
+ {
+ "name": "Indjedetet",
+ "pos_x": -19.625,
+ "pos_y": -137.96875,
+ "pos_z": -25.78125,
+ "stations": "Smoot Platform,Payne Relay"
+ },
+ {
+ "name": "Indjibandi",
+ "pos_x": 121.1875,
+ "pos_y": -71.75,
+ "pos_z": 107.03125,
+ "stations": "Takamizawa City,von Zach Port,Frobenius Landing,Bigourdan Dock"
+ },
+ {
+ "name": "Indjigan",
+ "pos_x": 0.9375,
+ "pos_y": -1.21875,
+ "pos_z": 109.90625,
+ "stations": "Nicollier Vision,Wisoff Prospect"
+ },
+ {
+ "name": "Indjin",
+ "pos_x": -116.125,
+ "pos_y": 11.96875,
+ "pos_z": 101,
+ "stations": "Needham's Claim,Hopkins Legacy"
+ },
+ {
+ "name": "Indra",
+ "pos_x": -60.65625,
+ "pos_y": -18.125,
+ "pos_z": -7.5,
+ "stations": "Wakata Dock,Currie Station,Ellis Dock,Eilenberg Terminal,Oramus Hub"
+ },
+ {
+ "name": "Indrachit",
+ "pos_x": 69.21875,
+ "pos_y": -116.21875,
+ "pos_z": 84.09375,
+ "stations": "Melotte Dock,Shirazi Orbital,Hickam Hub,Zarnecki Vision,Ibold Keep,Macdonald Holdings,Wood Port,Nomen Vision,Chertovsky Dock"
+ },
+ {
+ "name": "Indrajangu",
+ "pos_x": 95.1875,
+ "pos_y": 18,
+ "pos_z": -36.34375,
+ "stations": "Larson Dock,Sladek Plant"
+ },
+ {
+ "name": "Indramasci",
+ "pos_x": -50.25,
+ "pos_y": -6.15625,
+ "pos_z": 85.3125,
+ "stations": "Shepard Settlement,Hilbert Platform,Thomas Keep"
+ },
+ {
+ "name": "Indras",
+ "pos_x": 68.53125,
+ "pos_y": 9.3125,
+ "pos_z": 122.28125,
+ "stations": "Wakata Port,Kimbrough Station"
+ },
+ {
+ "name": "Indraseno",
+ "pos_x": -132.875,
+ "pos_y": 36.0625,
+ "pos_z": 17.3125,
+ "stations": "McMonagle Station,Murray Dock,Voss Dock,Burckhardt Installation"
+ },
+ {
+ "name": "Indrasians",
+ "pos_x": -95.71875,
+ "pos_y": -17.34375,
+ "pos_z": -16.96875,
+ "stations": "Carter Ring"
+ },
+ {
+ "name": "Indri",
+ "pos_x": -79.03125,
+ "pos_y": 65.90625,
+ "pos_z": -51.96875,
+ "stations": "Kanwar Works,Shelley Mines,Ingstad Orbital,Reed Landing,Jones Penal colony"
+ },
+ {
+ "name": "Indria",
+ "pos_x": 151.0625,
+ "pos_y": 7.78125,
+ "pos_z": 67.75,
+ "stations": "Hughes-Fulford Beacon,Kaku Camp"
+ },
+ {
+ "name": "Indrians",
+ "pos_x": -45.625,
+ "pos_y": 71.875,
+ "pos_z": 24.21875,
+ "stations": "Silves Port,Watts Dock,Herreshoff Point,Hill Station"
+ },
+ {
+ "name": "Indrundjara",
+ "pos_x": -54.8125,
+ "pos_y": -42.125,
+ "pos_z": 25.625,
+ "stations": "Cabana Hangar,Ellis Penal colony"
+ },
+ {
+ "name": "Ingaman",
+ "pos_x": 36.53125,
+ "pos_y": -38.25,
+ "pos_z": 109.15625,
+ "stations": "Hill Orbital,Judson Gateway,Yolen Landing"
+ },
+ {
+ "name": "Ingamba",
+ "pos_x": 140.40625,
+ "pos_y": -123.21875,
+ "pos_z": 16.1875,
+ "stations": "Marcy Vision,Hansteen Hub,Ayers Orbital,Humphreys Beacon,Koishikawa Base,Whitford Vision"
+ },
+ {
+ "name": "Ingariko",
+ "pos_x": 26.28125,
+ "pos_y": -84.25,
+ "pos_z": -102.375,
+ "stations": "Clervoy Platform,Schirra Prospect,Franklin Holdings,Whitson Point"
+ },
+ {
+ "name": "Inggale",
+ "pos_x": -46.1875,
+ "pos_y": -32.09375,
+ "pos_z": -17.59375,
+ "stations": "Whitney Station"
+ },
+ {
+ "name": "Inggarda",
+ "pos_x": 163.28125,
+ "pos_y": -91.9375,
+ "pos_z": 106.5625,
+ "stations": "Marriott Outpost"
+ },
+ {
+ "name": "Ingguromal",
+ "pos_x": -1.65625,
+ "pos_y": -66.0625,
+ "pos_z": 70.875,
+ "stations": "Spielberg Hub,Cogswell Hangar,Betancourt Plant,Nikitin Bastion"
+ },
+ {
+ "name": "Ingui",
+ "pos_x": 215.40625,
+ "pos_y": -171.09375,
+ "pos_z": 17.28125,
+ "stations": "Phillips Terminal,Schomburg Mines,Aitken Mines,Amano Installation"
+ },
+ {
+ "name": "Ingwaz",
+ "pos_x": 80.03125,
+ "pos_y": -109.09375,
+ "pos_z": 68.78125,
+ "stations": "Stromgren Arsenal,Check Hub,Sei Vision,Ilyushin Landing"
+ },
+ {
+ "name": "Inheimagni",
+ "pos_x": -60.8125,
+ "pos_y": -134.8125,
+ "pos_z": -17.9375,
+ "stations": "Morgan Terminal,Springer Gateway,Popov Hub,Soto Holdings,Linaweaver Plant"
+ },
+ {
+ "name": "Inherdis",
+ "pos_x": 49.15625,
+ "pos_y": -255.3125,
+ "pos_z": 72.4375,
+ "stations": "Flettner Dock,Fedden Colony,Bohrmann Camp"
+ },
+ {
+ "name": "Inheretii",
+ "pos_x": 141.0625,
+ "pos_y": -13.3125,
+ "pos_z": -40.40625,
+ "stations": "Hoften Enterprise,Mayer Base,Asimov Vision,Lucretius Station"
+ },
+ {
+ "name": "Inhers",
+ "pos_x": -24.84375,
+ "pos_y": -79.28125,
+ "pos_z": -64.71875,
+ "stations": "Tereshkova Hub,Hoyle Vision,Vonnegut Hub"
+ },
+ {
+ "name": "Inherseku",
+ "pos_x": -15.8125,
+ "pos_y": -110.9375,
+ "pos_z": -98.5625,
+ "stations": "Hart Beacon,Soddy Port"
+ },
+ {
+ "name": "Inherth",
+ "pos_x": 26.90625,
+ "pos_y": -167.84375,
+ "pos_z": -48.5,
+ "stations": "Ban Vision,Goryu Oasis,Westphal Prospect"
+ },
+ {
+ "name": "Ining",
+ "pos_x": -59.09375,
+ "pos_y": 56.25,
+ "pos_z": -78.5625,
+ "stations": "Shaara Terminal,Katzenstein Orbital,Gloss Terminal,Emshwiller Terminal,Riemann Settlement,Przhevalsky Enterprise,Bombelli Dock,Clark Enterprise,Carstensz Port,Bailey Enterprise,Gernsback Legacy"
+ },
+ {
+ "name": "Ining Dun",
+ "pos_x": 142.84375,
+ "pos_y": 8.625,
+ "pos_z": -40.03125,
+ "stations": "Bertin Refinery,Otto Platform,Howard Mine"
+ },
+ {
+ "name": "Inino",
+ "pos_x": 13.65625,
+ "pos_y": -4.46875,
+ "pos_z": 168.09375,
+ "stations": "Klink Dock,Burnham Survey,Dorsey Laboratory"
+ },
+ {
+ "name": "Ininohima",
+ "pos_x": 20.46875,
+ "pos_y": -103.84375,
+ "pos_z": -14.28125,
+ "stations": "Skjellerup Terminal"
+ },
+ {
+ "name": "Inktasa",
+ "pos_x": -36,
+ "pos_y": 57.90625,
+ "pos_z": 35.71875,
+ "stations": "Lovell Hub,Oleskiw Station,Huygens City,Reynolds Installation,Gregory Silo"
+ },
+ {
+ "name": "Inktehit",
+ "pos_x": 49,
+ "pos_y": -186.4375,
+ "pos_z": 102.84375,
+ "stations": "Karman Vision,Porco Port,Lemonnier Station,Clapperton Depot"
+ },
+ {
+ "name": "Inktomecane",
+ "pos_x": -59.03125,
+ "pos_y": 100.8125,
+ "pos_z": -103.625,
+ "stations": "Bulgakov Dock,Kuttner Camp"
+ },
+ {
+ "name": "Inmutha",
+ "pos_x": -46.6875,
+ "pos_y": -87.875,
+ "pos_z": 38.03125,
+ "stations": "Bujold Orbital,North Port,Mitropoulos City,Goonan Holdings,Abernathy Gateway,Herreshoff Enterprise,Ponce de Leon Hub,Banneker Point"
+ },
+ {
+ "name": "Inmutintien",
+ "pos_x": 82.375,
+ "pos_y": -82.03125,
+ "pos_z": -66.15625,
+ "stations": "Effinger Port,Francis Reach,Brule Mines,Wrangell Bastion"
+ },
+ {
+ "name": "Inovae",
+ "pos_x": -75.1875,
+ "pos_y": 127,
+ "pos_z": -7.8125,
+ "stations": "Ryman Station,Clark Settlement,Navigator Holdings,Aldiss Vision,Bates Lab"
+ },
+ {
+ "name": "Inovandar",
+ "pos_x": -80.46875,
+ "pos_y": -3.65625,
+ "pos_z": -83.28125,
+ "stations": "Smith Terminal,Moskowitz Beacon,Vasquez de Coronado's Inheritance,Laird Silo,Scortia's Progress"
+ },
+ {
+ "name": "Inovii",
+ "pos_x": -3.59375,
+ "pos_y": 36.75,
+ "pos_z": 118.28125,
+ "stations": "Lubin Installation,Fawcett Plant,Rozhdestvensky City"
+ },
+ {
+ "name": "Inovik",
+ "pos_x": 59.0625,
+ "pos_y": -15.15625,
+ "pos_z": -5.65625,
+ "stations": "Vaucanson Relay,Dias Ring,Gaspar de Lemos Hub,Bartlett Orbital,Oren Silo"
+ },
+ {
+ "name": "Inovit",
+ "pos_x": -103.0625,
+ "pos_y": 76.15625,
+ "pos_z": -61.09375,
+ "stations": "Archimedes Horizons"
+ },
+ {
+ "name": "Insubi",
+ "pos_x": 98.25,
+ "pos_y": 117.90625,
+ "pos_z": 48.9375,
+ "stations": "Sternberg Mine"
+ },
+ {
+ "name": "Insubii",
+ "pos_x": -85.40625,
+ "pos_y": 63.46875,
+ "pos_z": -88.78125,
+ "stations": "Leeuwenhoek Hub"
+ },
+ {
+ "name": "Intec",
+ "pos_x": 148.90625,
+ "pos_y": -56.125,
+ "pos_z": 30.125,
+ "stations": "Henson Survey"
+ },
+ {
+ "name": "Intener",
+ "pos_x": -56.875,
+ "pos_y": -8.9375,
+ "pos_z": -138.09375,
+ "stations": "Cleve Settlement,Gunn Colony,Chiang Works"
+ },
+ {
+ "name": "Intes",
+ "pos_x": 36.1875,
+ "pos_y": 122.125,
+ "pos_z": -99.59375,
+ "stations": "Tanner Horizons,Pauli Survey"
+ },
+ {
+ "name": "Inti",
+ "pos_x": -10.09375,
+ "pos_y": -40.75,
+ "pos_z": -36,
+ "stations": "Murray Hub,Kovalevsky Dock,Baturin Hub,Godwin Port,Artsebarsky Dock,Cori Dock,Sturckow Installation,Andreas Gateway,Noli Beacon,Napier Depot,Murdoch City,Reiter Ring,Feustel Observatory,Goeschke Settlement"
+ },
+ {
+ "name": "Inupiat",
+ "pos_x": 50.375,
+ "pos_y": 76.21875,
+ "pos_z": -62.375,
+ "stations": "Smeaton Landing,Ray Platform"
+ },
+ {
+ "name": "Inupiates",
+ "pos_x": 70.875,
+ "pos_y": -74,
+ "pos_z": 32.15625,
+ "stations": "Ashman Station,Rankin Terminal,Jordan's Claim,Wolszczan Terminal"
+ },
+ {
+ "name": "Inupinie",
+ "pos_x": 137.53125,
+ "pos_y": -140.03125,
+ "pos_z": 59.53125,
+ "stations": "Baillaud Keep,Naburimannu Survey,Schwabe Vision"
+ },
+ {
+ "name": "Iota Aquarii",
+ "pos_x": -78.875,
+ "pos_y": -133.90625,
+ "pos_z": 80.84375,
+ "stations": "Miyasaka Dock"
+ },
+ {
+ "name": "Iota Crucis",
+ "pos_x": 101.09375,
+ "pos_y": 3.375,
+ "pos_z": 64.625,
+ "stations": "Lawson Enterprise,Drebbel Terminal"
+ },
+ {
+ "name": "Iota Eridani",
+ "pos_x": 61.90625,
+ "pos_y": -135.1875,
+ "pos_z": -24.34375,
+ "stations": "Forfait Landing,Fowler Survey,Hipparchus Terminal"
+ },
+ {
+ "name": "Iota Horologii",
+ "pos_x": 29.4375,
+ "pos_y": -47.625,
+ "pos_z": -0.9375,
+ "stations": "Egan Penal colony,Bernard Colony,Cabrera Prospect,Bessemer Station,Dirac Refinery"
+ },
+ {
+ "name": "Iota Hydri",
+ "pos_x": 70.75,
+ "pos_y": -58.59375,
+ "pos_z": 30.84375,
+ "stations": "Iben Hub,Szameit Barracks,Babcock Vision,Mikoyan Beacon,Pavlou Prospect"
+ },
+ {
+ "name": "Iota Pavonis",
+ "pos_x": 25,
+ "pos_y": -19.34375,
+ "pos_z": 47.9375,
+ "stations": "Delany City,Koontz Base"
+ },
+ {
+ "name": "Iota Persei",
+ "pos_x": -19.59375,
+ "pos_y": -4.15625,
+ "pos_z": -27.96875,
+ "stations": "Walker City"
+ },
+ {
+ "name": "Iota Pictoris A",
+ "pos_x": 92.75,
+ "pos_y": -76.21875,
+ "pos_z": -14.46875,
+ "stations": "Barmin Dock,Schiaparelli Prospect,Faber Installation,Johnson Laboratory"
+ },
+ {
+ "name": "Iota Pictoris B",
+ "pos_x": 96.40625,
+ "pos_y": -79.21875,
+ "pos_z": -15.03125,
+ "stations": "Ryle Base"
+ },
+ {
+ "name": "Iota Piscium",
+ "pos_x": -26.875,
+ "pos_y": -35.75,
+ "pos_z": -1.71875,
+ "stations": "Virts City"
+ },
+ {
+ "name": "Iota Trianguli Australis",
+ "pos_x": 72.4375,
+ "pos_y": -24,
+ "pos_z": 100.9375,
+ "stations": "Efremov Dock,Shapiro Plant,Ambartsumian Prospect"
+ },
+ {
+ "name": "Iota Tucanae",
+ "pos_x": 150.5,
+ "pos_y": -250.71875,
+ "pos_z": 84.03125,
+ "stations": "Barr Mines,Tanaka Vision,Rigaux Colony"
+ },
+ {
+ "name": "Iota-1 Normae",
+ "pos_x": 76.8125,
+ "pos_y": -10.75,
+ "pos_z": 116.78125,
+ "stations": "Zhen Port,Curie Settlement"
+ },
+ {
+ "name": "Iota-2 Fornacis",
+ "pos_x": 32.5625,
+ "pos_y": -101.125,
+ "pos_z": -31.25,
+ "stations": "Bosch Horizons,Daniel Holdings"
+ },
+ {
+ "name": "Ipet",
+ "pos_x": 88.71875,
+ "pos_y": -16.5,
+ "pos_z": -47.46875,
+ "stations": "Meitner City,Galilei Ring"
+ },
+ {
+ "name": "Ipetae",
+ "pos_x": -122.0625,
+ "pos_y": -74.34375,
+ "pos_z": 5.59375,
+ "stations": "Wheelock Landing,Wellman Vision,Alcala Station"
+ },
+ {
+ "name": "Ipete",
+ "pos_x": 162.6875,
+ "pos_y": 8.09375,
+ "pos_z": 21.75,
+ "stations": "Kondakova Prospect,Hernandez Stop"
+ },
+ {
+ "name": "Ipeterai",
+ "pos_x": -57.28125,
+ "pos_y": 169.09375,
+ "pos_z": -30,
+ "stations": "Crown Camp,Williamson Horizons"
+ },
+ {
+ "name": "Ipetes",
+ "pos_x": -4.28125,
+ "pos_y": 29.28125,
+ "pos_z": 95.78125,
+ "stations": "Liebig Survey"
+ },
+ {
+ "name": "Ipila",
+ "pos_x": 145.75,
+ "pos_y": 60.9375,
+ "pos_z": -6.1875,
+ "stations": "Williamson Settlement"
+ },
+ {
+ "name": "Ipilars",
+ "pos_x": -115.46875,
+ "pos_y": 117.1875,
+ "pos_z": 106.90625,
+ "stations": "Hambly Depot"
+ },
+ {
+ "name": "Ipillke",
+ "pos_x": -77.90625,
+ "pos_y": 11.0625,
+ "pos_z": 27.3125,
+ "stations": "Bulleid Dock,Chapman's Folly"
+ },
+ {
+ "name": "Ipilyak",
+ "pos_x": 80.9375,
+ "pos_y": 29.6875,
+ "pos_z": 30.15625,
+ "stations": "Sakers Outpost,Jeschke Dock"
+ },
+ {
+ "name": "Ipilyaqa",
+ "pos_x": 70.96875,
+ "pos_y": -134.625,
+ "pos_z": 53.1875,
+ "stations": "Furuta Orbital,Bracewell Ring,Rolland Market"
+ },
+ {
+ "name": "Iptera",
+ "pos_x": 76.625,
+ "pos_y": 101.5625,
+ "pos_z": 41.09375,
+ "stations": "Dunbar Beacon"
+ },
+ {
+ "name": "Ipterod",
+ "pos_x": -63.0625,
+ "pos_y": -45.84375,
+ "pos_z": 112.78125,
+ "stations": "Vinge Vision,Asprin Terminal,Friesner Prospect,Phillpotts Town"
+ },
+ {
+ "name": "Iptet",
+ "pos_x": 21.875,
+ "pos_y": -201.78125,
+ "pos_z": 60.84375,
+ "stations": "Helffrich Ring,Villarceau Vision"
+ },
+ {
+ "name": "Iranadhisa",
+ "pos_x": 12.0625,
+ "pos_y": 116.96875,
+ "pos_z": 28.375,
+ "stations": "Corte-Real Market,Kingsmill Port,Lefschetz Orbital,Haldeman Dock"
+ },
+ {
+ "name": "Irandan",
+ "pos_x": -86.375,
+ "pos_y": -12.40625,
+ "pos_z": -125.0625,
+ "stations": "Glazkov Terminal,Binnie Ring,Disch Arena,Guerrero Camp,Rescue Ship - Glazkov Terminal"
+ },
+ {
+ "name": "Irantxe",
+ "pos_x": -53.1875,
+ "pos_y": -149.4375,
+ "pos_z": 71.5625,
+ "stations": "Carsono Landing"
+ },
+ {
+ "name": "Irdlivices",
+ "pos_x": -8.84375,
+ "pos_y": 57.125,
+ "pos_z": -115.34375,
+ "stations": "Maine Terminal,Irwin Plant,Zoline Station,Paez Hub,Guidoni Point"
+ },
+ {
+ "name": "Irdlivuni",
+ "pos_x": 167.0625,
+ "pos_y": -78.1875,
+ "pos_z": 69.4375,
+ "stations": "Zarnecki Point,Kapteyn Vision"
+ },
+ {
+ "name": "Irukaiabozo",
+ "pos_x": 86.28125,
+ "pos_y": -212.46875,
+ "pos_z": -34.71875,
+ "stations": "Suri Ring,Pond Penal colony,McDermott Port,Faye Hub,Shipton Beacon,Stjepan Seljan Terminal"
+ },
+ {
+ "name": "Irukama",
+ "pos_x": 140.71875,
+ "pos_y": -96.96875,
+ "pos_z": 67.78125,
+ "stations": "Blaauw City,Crossfield Orbital,Serre Survey,Fadlan Barracks"
+ },
+ {
+ "name": "Irukami",
+ "pos_x": 60.875,
+ "pos_y": 105.53125,
+ "pos_z": 23.75,
+ "stations": "Lanchester City,Webb's Claim,Xiaoguan Bastion"
+ },
+ {
+ "name": "Irukan",
+ "pos_x": 26.1875,
+ "pos_y": 104.46875,
+ "pos_z": -131.0625,
+ "stations": "Tennyson d'Eyncourt Point,King's Claim"
+ },
+ {
+ "name": "Irukas",
+ "pos_x": 161.84375,
+ "pos_y": -125.5,
+ "pos_z": -50.21875,
+ "stations": "Wirtanen's Claim,Sikorsky Survey,Macedo Base"
+ },
+ {
+ "name": "Irula",
+ "pos_x": 118.8125,
+ "pos_y": 3.71875,
+ "pos_z": -2.4375,
+ "stations": "Anderson Orbital,Egan Relay,Houtman Prospect"
+ },
+ {
+ "name": "Irulachan",
+ "pos_x": -41.65625,
+ "pos_y": -61.59375,
+ "pos_z": 81.8125,
+ "stations": "Sevastyanov Survey,von Helmont Depot,Soddy Asylum"
+ },
+ {
+ "name": "Iruli",
+ "pos_x": 20.46875,
+ "pos_y": -102.59375,
+ "pos_z": 163.59375,
+ "stations": "Fabian Orbital,Pogson Port"
+ },
+ {
+ "name": "Irulis",
+ "pos_x": -104.59375,
+ "pos_y": -9.40625,
+ "pos_z": -57.25,
+ "stations": "Collins Refinery,Boswell Dock,Babbage Terminal,Chilton Base"
+ },
+ {
+ "name": "Irusamara",
+ "pos_x": -11.25,
+ "pos_y": -164.75,
+ "pos_z": -62.375,
+ "stations": "Schmidt Survey,Vasquez de Coronado Settlement,Swift Horizons"
+ },
+ {
+ "name": "Irusan",
+ "pos_x": 42.8125,
+ "pos_y": -41.21875,
+ "pos_z": -135.8125,
+ "stations": "Onufriyenko Port,Bella Port"
+ },
+ {
+ "name": "Irush",
+ "pos_x": 100.1875,
+ "pos_y": -139.09375,
+ "pos_z": 121.53125,
+ "stations": "Digges Depot"
+ },
+ {
+ "name": "Ishtar",
+ "pos_x": 6.75,
+ "pos_y": 21.5625,
+ "pos_z": -53,
+ "stations": "Vinci Base"
+ },
+ {
+ "name": "Isinor",
+ "pos_x": 67.125,
+ "pos_y": 68.1875,
+ "pos_z": 54.96875,
+ "stations": "Charnas Terminal"
+ },
+ {
+ "name": "Isis",
+ "pos_x": 11.5625,
+ "pos_y": -60.53125,
+ "pos_z": 26.5625,
+ "stations": "Schwarzschild Stadium,Heaviside Arsenal,Levchenko Relay"
+ },
+ {
+ "name": "Isita",
+ "pos_x": -78.9375,
+ "pos_y": 20.78125,
+ "pos_z": 117.21875,
+ "stations": "Xing Terminal,Pauli's Claim"
+ },
+ {
+ "name": "Isitab",
+ "pos_x": 67.1875,
+ "pos_y": -78.0625,
+ "pos_z": 118.34375,
+ "stations": "Dias Observatory,Webb Arsenal,Bowen Platform"
+ },
+ {
+ "name": "Isitani",
+ "pos_x": -9.25,
+ "pos_y": 35.9375,
+ "pos_z": -155.78125,
+ "stations": "Creamer Ring,Dorsett Port,Goddard Dock,Hughes-Fulford Survey"
+ },
+ {
+ "name": "Isiti",
+ "pos_x": 73.375,
+ "pos_y": -203.78125,
+ "pos_z": 100.875,
+ "stations": "Altuna Port,Perrine Port,Harvia Orbital,Watts Landing"
+ },
+ {
+ "name": "Isitou",
+ "pos_x": -39.53125,
+ "pos_y": 14.9375,
+ "pos_z": 123.03125,
+ "stations": "Riemann Dock"
+ },
+ {
+ "name": "Isla",
+ "pos_x": -140.8125,
+ "pos_y": -84.25,
+ "pos_z": -32.0625,
+ "stations": "Alvares Gateway,Turtledove Terminal,Felice Port,Herreshoff's Folly,Dorsey Depot"
+ },
+ {
+ "name": "Islasto",
+ "pos_x": 60.28125,
+ "pos_y": 47.8125,
+ "pos_z": 12.34375,
+ "stations": "Brunel Prospect,Teng-hui Point"
+ },
+ {
+ "name": "Islastyara",
+ "pos_x": -35.1875,
+ "pos_y": -7.4375,
+ "pos_z": 91,
+ "stations": "Levinson Relay,Broderick Refinery"
+ },
+ {
+ "name": "Isleta",
+ "pos_x": 3.6875,
+ "pos_y": -2.34375,
+ "pos_z": 42.625,
+ "stations": "Olsen Station,Ivanov Enterprise,Atwater Dock,Shinn's Inheritance,Palmer's Progress"
+ },
+ {
+ "name": "Istanu",
+ "pos_x": 2.0625,
+ "pos_y": 55.15625,
+ "pos_z": 1.28125,
+ "stations": "Younghusband Point,Haber Prospect"
+ },
+ {
+ "name": "Itela",
+ "pos_x": -20.25,
+ "pos_y": 8.03125,
+ "pos_z": 83.5,
+ "stations": "Deere Landing,Hawking Prospect"
+ },
+ {
+ "name": "Iteliuona",
+ "pos_x": -120.78125,
+ "pos_y": -81.5,
+ "pos_z": -10.84375,
+ "stations": "Krylov Hub,Steiner City,Navigator Port,Obruchev Point"
+ },
+ {
+ "name": "Itelmasa",
+ "pos_x": 207.78125,
+ "pos_y": -113.71875,
+ "pos_z": 5.8125,
+ "stations": "Hirn Dock,Berkey Vision,Metz Silo"
+ },
+ {
+ "name": "Itelmen",
+ "pos_x": 196.21875,
+ "pos_y": -139.28125,
+ "pos_z": -23.9375,
+ "stations": "Fukushima Works,Boyajian Colony"
+ },
+ {
+ "name": "Itelmens",
+ "pos_x": 101.59375,
+ "pos_y": -62.09375,
+ "pos_z": 92.8125,
+ "stations": "Ashbrook Vision"
+ },
+ {
+ "name": "Ithaca",
+ "pos_x": -8.09375,
+ "pos_y": 44.9375,
+ "pos_z": -9.28125,
+ "stations": "Hume Depot,Pohl Keep"
+ },
+ {
+ "name": "Ithel",
+ "pos_x": 188.40625,
+ "pos_y": -180.46875,
+ "pos_z": 59.84375,
+ "stations": "Anderson Colony,Russell Base"
+ },
+ {
+ "name": "Ithelki",
+ "pos_x": 46.625,
+ "pos_y": -151.71875,
+ "pos_z": -64.9375,
+ "stations": "Hoerner Platform,Wang Prospect,Binder Refinery"
+ },
+ {
+ "name": "Ithentia",
+ "pos_x": -7.28125,
+ "pos_y": 25.46875,
+ "pos_z": 146.34375,
+ "stations": "Lavoisier Dock"
+ },
+ {
+ "name": "Ither",
+ "pos_x": -112.25,
+ "pos_y": 15,
+ "pos_z": 89.3125,
+ "stations": "Aleksandrov Colony,Wohler Camp,Thompson Holdings,Dunn Installation"
+ },
+ {
+ "name": "Ithertrudhr",
+ "pos_x": 50.875,
+ "pos_y": -203.15625,
+ "pos_z": -30.78125,
+ "stations": "Bailly Point"
+ },
+ {
+ "name": "Ithurs",
+ "pos_x": 142.625,
+ "pos_y": -150.625,
+ "pos_z": 119.84375,
+ "stations": "Oterma Works,Bullialdus Vision,Abe Installation,Reichelt Beacon"
+ },
+ {
+ "name": "Itototangi",
+ "pos_x": 63.28125,
+ "pos_y": -53.5625,
+ "pos_z": -59.4375,
+ "stations": "Shatalov Refinery,Deere Hub"
+ },
+ {
+ "name": "Itza",
+ "pos_x": -29.65625,
+ "pos_y": -14.125,
+ "pos_z": -67.6875,
+ "stations": "Ellison Station,Hennepin Enterprise,Ore Terminal,Cochrane Terminal,Luiken Port,Froude Station,Fontenay Orbital,McDonald Port,Shelley Landing,McDaniel Station,Murphy's Claim"
+ },
+ {
+ "name": "Itzam Cimi",
+ "pos_x": -7.625,
+ "pos_y": -121.375,
+ "pos_z": 78.96875,
+ "stations": "Phillips' Claim"
+ },
+ {
+ "name": "Itzam Crua",
+ "pos_x": 138.28125,
+ "pos_y": -117.75,
+ "pos_z": -23.46875,
+ "stations": "Joy Station,Yize Survey,Phillips Silo"
+ },
+ {
+ "name": "Itzam Nara",
+ "pos_x": -55.875,
+ "pos_y": 7.5,
+ "pos_z": 156.25,
+ "stations": "Frechet Dock,Fowler Settlement,Irens Survey"
+ },
+ {
+ "name": "Itzam Nawe",
+ "pos_x": 93.1875,
+ "pos_y": 143.875,
+ "pos_z": 36.5,
+ "stations": "Zettel Enterprise,Britnev Observatory,Hedley Hub"
+ },
+ {
+ "name": "Itzambivat",
+ "pos_x": -111.15625,
+ "pos_y": 8.75,
+ "pos_z": 71.25,
+ "stations": "Grzimek Stop,Rutherford Installation"
+ },
+ {
+ "name": "Itzamnet",
+ "pos_x": 36.125,
+ "pos_y": -160.96875,
+ "pos_z": 156.15625,
+ "stations": "Seamans' Claim,Pons Dock"
+ },
+ {
+ "name": "Itzamnets",
+ "pos_x": -48.03125,
+ "pos_y": -146.65625,
+ "pos_z": 65.0625,
+ "stations": "Bowell Port,Heinlein Depot,Shajn Terminal,Roelofs Terminal"
+ },
+ {
+ "name": "Itzamni",
+ "pos_x": 143.78125,
+ "pos_y": -73.125,
+ "pos_z": -60.65625,
+ "stations": "Seitter Landing,Hoyle Colony,Ludwig Struve Camp"
+ },
+ {
+ "name": "Itzani",
+ "pos_x": 38.65625,
+ "pos_y": 46.5625,
+ "pos_z": 63.53125,
+ "stations": "Stafford Refinery,Piper Survey,Jacquard City"
+ },
+ {
+ "name": "Itzanii",
+ "pos_x": 17.5625,
+ "pos_y": 93.625,
+ "pos_z": 80.375,
+ "stations": "Sladek Dock,Bendell Platform"
+ },
+ {
+ "name": "IV Comae Berenices",
+ "pos_x": 21.6875,
+ "pos_y": 90.53125,
+ "pos_z": -3.53125,
+ "stations": "Litke Dock,Gotlieb Base"
+ },
+ {
+ "name": "Iverdi",
+ "pos_x": 126.625,
+ "pos_y": -33.59375,
+ "pos_z": -103.46875,
+ "stations": "Madsen Landing,Phillips Platform"
+ },
+ {
+ "name": "Iveri",
+ "pos_x": -100.3125,
+ "pos_y": -83.9375,
+ "pos_z": 119.15625,
+ "stations": "Lerman Landing,Darnielle Colony"
+ },
+ {
+ "name": "Iverni",
+ "pos_x": -141.15625,
+ "pos_y": 76.9375,
+ "pos_z": -20.3125,
+ "stations": "Al-Jazari Refinery,Mieville Installation,Arkwright Refinery"
+ },
+ {
+ "name": "Ivernten",
+ "pos_x": -67.96875,
+ "pos_y": 91.90625,
+ "pos_z": 160.34375,
+ "stations": "Wilder Sanctuary,Wisniewski-Snerg Relay,Rond d'Alembert Base"
+ },
+ {
+ "name": "Iwaidja",
+ "pos_x": 16.40625,
+ "pos_y": -239.40625,
+ "pos_z": -12.65625,
+ "stations": "Messerschmitt Port,Lee Enterprise"
+ },
+ {
+ "name": "Ix",
+ "pos_x": -65.21875,
+ "pos_y": 7.75,
+ "pos_z": -111.03125,
+ "stations": "Scully-Power Station,Walker Depot,Diesel's Inheritance"
+ },
+ {
+ "name": "Ix Chakan",
+ "pos_x": -13.0625,
+ "pos_y": -43.65625,
+ "pos_z": 55.3125,
+ "stations": "Bennett Enterprise,Lebedev Enterprise"
+ },
+ {
+ "name": "Ix Chau",
+ "pos_x": -84.9375,
+ "pos_y": -82.0625,
+ "pos_z": -115.125,
+ "stations": "Sabine Prospect"
+ },
+ {
+ "name": "Ix Chim",
+ "pos_x": -78.71875,
+ "pos_y": -5.84375,
+ "pos_z": -79.21875,
+ "stations": "Crippen Mines,Velazquez Vision,Farkas Ring,Galton Landing,Cartan Settlement"
+ },
+ {
+ "name": "Ix Ching",
+ "pos_x": 33.34375,
+ "pos_y": -78.15625,
+ "pos_z": 171.8125,
+ "stations": "Fan Mines,Palitzsch Colony,Puleston Vision"
+ },
+ {
+ "name": "Ix Chita",
+ "pos_x": -14.21875,
+ "pos_y": -55.3125,
+ "pos_z": 141.25,
+ "stations": "Isherwood Station,Jones Point,Dietz Base,Bradley Orbital"
+ },
+ {
+ "name": "Ix Chodharr",
+ "pos_x": -163.3125,
+ "pos_y": 51.71875,
+ "pos_z": -96.34375,
+ "stations": "Peirce Orbital,Haber's Exile"
+ },
+ {
+ "name": "Ix Chons",
+ "pos_x": 94.46875,
+ "pos_y": -173.53125,
+ "pos_z": 1.84375,
+ "stations": "Carpenter Orbital,Yanai Mines,Stein Depot"
+ },
+ {
+ "name": "Ix Chowagit",
+ "pos_x": 122.625,
+ "pos_y": -160.5,
+ "pos_z": 47.40625,
+ "stations": "Oterma City,Christy Orbital,Challis Station,Patry Orbital,Wilhelm Klinkerfues Port,McDermott Ring,Howard Plant,Mohr City"
+ },
+ {
+ "name": "Ix Chuacae",
+ "pos_x": -14.90625,
+ "pos_y": -51.96875,
+ "pos_z": 140.65625,
+ "stations": "Williams Beacon,Ashton Outpost,Sauma Hangar,Szameit Colony"
+ },
+ {
+ "name": "Ix Chuntin",
+ "pos_x": 117.25,
+ "pos_y": -79.4375,
+ "pos_z": 122.84375,
+ "stations": "Charlois Outpost"
+ },
+ {
+ "name": "Ix Chup",
+ "pos_x": 96.71875,
+ "pos_y": -180.625,
+ "pos_z": 93.4375,
+ "stations": "Mizuno Vision,Brunel Bastion"
+ },
+ {
+ "name": "Ix Tub Tun",
+ "pos_x": 10.9375,
+ "pos_y": -178.15625,
+ "pos_z": -13.46875,
+ "stations": "Shaw Base,Rogers Hub"
+ },
+ {
+ "name": "Ix Tun",
+ "pos_x": -32.65625,
+ "pos_y": 83.84375,
+ "pos_z": 61.15625,
+ "stations": "Reed Hangar,Taylor Settlement"
+ },
+ {
+ "name": "Ixbakah",
+ "pos_x": 3.75,
+ "pos_y": -144.53125,
+ "pos_z": -67,
+ "stations": "Stein Retreat"
+ },
+ {
+ "name": "Ixbakald",
+ "pos_x": 81.25,
+ "pos_y": -93.125,
+ "pos_z": -39.28125,
+ "stations": "Hanke-Woods Orbital,Miletus Relay"
+ },
+ {
+ "name": "Ixbaksha",
+ "pos_x": -146.03125,
+ "pos_y": -39.65625,
+ "pos_z": -20.53125,
+ "stations": "Galiano's Claim,Yolen Colony"
+ },
+ {
+ "name": "Ixbalan",
+ "pos_x": 87.8125,
+ "pos_y": -81.34375,
+ "pos_z": -136.25,
+ "stations": "Teng Port,Andree Survey"
+ },
+ {
+ "name": "Ixbalanque",
+ "pos_x": 77.5,
+ "pos_y": -55.40625,
+ "pos_z": -96.8125,
+ "stations": "Soto Stop,Cardano Sanctuary"
+ },
+ {
+ "name": "Ixcateshai",
+ "pos_x": 96.34375,
+ "pos_y": -120.25,
+ "pos_z": -62.40625,
+ "stations": "Lockyer Depot,Lagrange Barracks"
+ },
+ {
+ "name": "Ixchertha",
+ "pos_x": 3.21875,
+ "pos_y": -97.375,
+ "pos_z": 88.3125,
+ "stations": "Sweet City,Struve Terminal"
+ },
+ {
+ "name": "Ixil",
+ "pos_x": -22.03125,
+ "pos_y": -183,
+ "pos_z": 68,
+ "stations": "Wilhelm von Struve Port,Conklin Prospect"
+ },
+ {
+ "name": "Ixilak",
+ "pos_x": 71.53125,
+ "pos_y": -173.5,
+ "pos_z": 154.53125,
+ "stations": "Nomen Platform,De Port,Frechet Installation"
+ },
+ {
+ "name": "Ixilalana",
+ "pos_x": 57.25,
+ "pos_y": 155.09375,
+ "pos_z": 37.15625,
+ "stations": "Weyl Vision,Pelliot Settlement,Jordan Dock,Stillman Hub,Francisco de Almeida Base"
+ },
+ {
+ "name": "Ixilalonta",
+ "pos_x": 131.71875,
+ "pos_y": -9.5,
+ "pos_z": -1.46875,
+ "stations": "Clairaut Installation,Mitchell Port,Nikitin Hub,Wyeth Port"
+ },
+ {
+ "name": "Ixtabru",
+ "pos_x": 124.28125,
+ "pos_y": -239.625,
+ "pos_z": -21,
+ "stations": "Hill Tinsley Mines"
+ },
+ {
+ "name": "Iyaka",
+ "pos_x": -23.46875,
+ "pos_y": 80.96875,
+ "pos_z": 45.78125,
+ "stations": "Giles Landing,Barth Prospect,de Sousa Terminal"
+ },
+ {
+ "name": "Iyakajauja",
+ "pos_x": -85.46875,
+ "pos_y": -144.625,
+ "pos_z": 62.6875,
+ "stations": "Beliaev Installation,Barr Plant,King Terminal"
+ },
+ {
+ "name": "Iyati",
+ "pos_x": 38.5625,
+ "pos_y": 130.21875,
+ "pos_z": -29.03125,
+ "stations": "Noli Dock,Steele Dock,Montrose Port"
+ },
+ {
+ "name": "Iyatiku",
+ "pos_x": -66.9375,
+ "pos_y": 128.5625,
+ "pos_z": -62.65625,
+ "stations": "Ehrenfried Kegel Settlement"
+ },
+ {
+ "name": "Iyatio",
+ "pos_x": -93.3125,
+ "pos_y": 36.0625,
+ "pos_z": 126.4375,
+ "stations": "Low Survey,Goonan Mines,Lewitt Relay"
+ },
+ {
+ "name": "Iyatsyapori",
+ "pos_x": -62.5625,
+ "pos_y": -149.34375,
+ "pos_z": 65.90625,
+ "stations": "van der Riet Woolley Landing"
+ },
+ {
+ "name": "Izanagi",
+ "pos_x": 45.4375,
+ "pos_y": -19.15625,
+ "pos_z": -8.8125,
+ "stations": "Piper Port,Wilcutt Hub,Manakov Ring,Grassmann Installation,Cartwright Terminal,McKee Beacon,Melvin Installation,Pasteur Port,Fincke Hub,Chadwick Hub"
+ },
+ {
+ "name": "Izho",
+ "pos_x": 46.53125,
+ "pos_y": 164.84375,
+ "pos_z": 23.75,
+ "stations": "Archer Settlement,Soto Mines"
+ },
+ {
+ "name": "Izhorians",
+ "pos_x": 99.5625,
+ "pos_y": 56.21875,
+ "pos_z": 56.75,
+ "stations": "Illy Platform,Shatalov Survey"
+ },
+ {
+ "name": "Jaadwadlia",
+ "pos_x": -69.46875,
+ "pos_y": 21.9375,
+ "pos_z": 79.28125,
+ "stations": "Hovell Hub,McCaffrey's Folly,Stabenow Survey,Taylor Enterprise"
+ },
+ {
+ "name": "Jaaka",
+ "pos_x": 120.71875,
+ "pos_y": -83.28125,
+ "pos_z": 108.4375,
+ "stations": "Amedeo Plana Orbital,al-Khowarizmi Vision,Shinn Installation,Anderson Port"
+ },
+ {
+ "name": "Jaakari",
+ "pos_x": -1.4375,
+ "pos_y": 92.625,
+ "pos_z": -116.84375,
+ "stations": "Haxel Orbital,Torricelli Terminal,Shepard Enterprise,Thornycroft Prospect,Cavendish's Inheritance"
+ },
+ {
+ "name": "Jaara",
+ "pos_x": 158.46875,
+ "pos_y": -18.875,
+ "pos_z": 57.21875,
+ "stations": "Asher Platform,Gabriel Relay"
+ },
+ {
+ "name": "Jaarn",
+ "pos_x": -23.3125,
+ "pos_y": -157,
+ "pos_z": 113.15625,
+ "stations": "Arend Vision,Araki Colony"
+ },
+ {
+ "name": "Jabaei",
+ "pos_x": -123.90625,
+ "pos_y": -10.15625,
+ "pos_z": 70.125,
+ "stations": "Alvares Base,So-yeon Orbital,Gillekens Station,Furukawa's Folly"
+ },
+ {
+ "name": "Jabaja",
+ "pos_x": 72.03125,
+ "pos_y": -243.5,
+ "pos_z": -22.53125,
+ "stations": "Busemann's Pride,Hardy's Exile"
+ },
+ {
+ "name": "Jabueyen",
+ "pos_x": 51.9375,
+ "pos_y": -151.3125,
+ "pos_z": 145.71875,
+ "stations": "Giraud Installation,Auzout Terminal,Kaiser's Claim"
+ },
+ {
+ "name": "Jabugaly",
+ "pos_x": 106.40625,
+ "pos_y": -146.59375,
+ "pos_z": -70.90625,
+ "stations": "van der Riet Woolley Orbital,Brashear Landing,Dampier's Progress"
+ },
+ {
+ "name": "Jabunnut",
+ "pos_x": -132,
+ "pos_y": -34.5,
+ "pos_z": 81.21875,
+ "stations": "Sagan Terminal,Jeschke Horizons,Verrazzano Terminal,Killough Enterprise,Dall Depot"
+ },
+ {
+ "name": "Jaburara",
+ "pos_x": 32.25,
+ "pos_y": -35.21875,
+ "pos_z": -7,
+ "stations": "Chadwick Station,Reynolds Hub,Franke Vision,Hausdorff Prospect"
+ },
+ {
+ "name": "Jaburouri",
+ "pos_x": -62.625,
+ "pos_y": 151.125,
+ "pos_z": 31.375,
+ "stations": "Meyrink Platform,McKay Camp"
+ },
+ {
+ "name": "Jadikin",
+ "pos_x": 88.6875,
+ "pos_y": 94.875,
+ "pos_z": -87.46875,
+ "stations": "Viktorenko Settlement,Sterling Survey"
+ },
+ {
+ "name": "Jadikinon",
+ "pos_x": 104.40625,
+ "pos_y": -202.5,
+ "pos_z": 3.03125,
+ "stations": "DiFate Terminal,Tago Vision,Reis Landing,Johri Terminal,Joseph Delambre Terminal,Dixon Station,Boss Dock,Honda Lab,Cerulli's Inheritance,Cook Barracks"
+ },
+ {
+ "name": "Jadimo",
+ "pos_x": -43.625,
+ "pos_y": -103.75,
+ "pos_z": 18.125,
+ "stations": "Grzimek Dock"
+ },
+ {
+ "name": "Jadliaura",
+ "pos_x": 35.875,
+ "pos_y": -57.125,
+ "pos_z": 104.96875,
+ "stations": "Murdoch's Progress,Stanley Estate,Lorrah Vision"
+ },
+ {
+ "name": "Jadlili",
+ "pos_x": -122.375,
+ "pos_y": 11.625,
+ "pos_z": 108.9375,
+ "stations": "Zewail Hub,Solovyev Horizons,Klink Prospect,Garnier Silo"
+ },
+ {
+ "name": "Jadlillke",
+ "pos_x": -59.53125,
+ "pos_y": -21.46875,
+ "pos_z": -55.65625,
+ "stations": "Elvstrom Ring,Markham Orbital"
+ },
+ {
+ "name": "Jadlir",
+ "pos_x": 136.5,
+ "pos_y": -61.03125,
+ "pos_z": -0.53125,
+ "stations": "Laurent Refinery,Millosevich Holdings"
+ },
+ {
+ "name": "Jafenhar",
+ "pos_x": 43.40625,
+ "pos_y": -227.6875,
+ "pos_z": 19.53125,
+ "stations": "Dreyer Relay"
+ },
+ {
+ "name": "Jafnharas",
+ "pos_x": -12.25,
+ "pos_y": -42.875,
+ "pos_z": 138.90625,
+ "stations": "Grimwood Camp,Sagan Dock,Hovell Enterprise"
+ },
+ {
+ "name": "Jafnhau",
+ "pos_x": -39.59375,
+ "pos_y": -77.65625,
+ "pos_z": -48.375,
+ "stations": "Battuta Port,Oppenheimer Hub,Slade Depot"
+ },
+ {
+ "name": "Jagai",
+ "pos_x": 59.90625,
+ "pos_y": -240.8125,
+ "pos_z": 63.625,
+ "stations": "Seyfert Colony,Merrill Port"
+ },
+ {
+ "name": "Jagaluk",
+ "pos_x": 130.3125,
+ "pos_y": -215.84375,
+ "pos_z": -24.125,
+ "stations": "Young Port,King Hub"
+ },
+ {
+ "name": "Jagantjin",
+ "pos_x": -71.96875,
+ "pos_y": -55.28125,
+ "pos_z": -98.34375,
+ "stations": "Burkin Dock,Britnev Depot"
+ },
+ {
+ "name": "Jagga",
+ "pos_x": 50,
+ "pos_y": -174.3125,
+ "pos_z": 150.125,
+ "stations": "Al-Kashi Penal colony,Adams Platform,Stein Station,Regiomontanus Survey"
+ },
+ {
+ "name": "Jaitic",
+ "pos_x": -100.78125,
+ "pos_y": 31.375,
+ "pos_z": 21.875,
+ "stations": "Nakaya Legacy"
+ },
+ {
+ "name": "Jaitrics",
+ "pos_x": -7.84375,
+ "pos_y": -171.34375,
+ "pos_z": 100.8125,
+ "stations": "Jung Survey,Coulomb Silo"
+ },
+ {
+ "name": "Jaitu",
+ "pos_x": -102.15625,
+ "pos_y": 21.75,
+ "pos_z": -17.3125,
+ "stations": "Wetherbee Hangar,Hughes Holdings,Novitski Refinery"
+ },
+ {
+ "name": "Jaityata",
+ "pos_x": -18.46875,
+ "pos_y": 94.03125,
+ "pos_z": 44.78125,
+ "stations": "Mayer Installation,Lorenz Horizons,Kopra Landing"
+ },
+ {
+ "name": "Jakab",
+ "pos_x": -133.9375,
+ "pos_y": 13.59375,
+ "pos_z": 74.1875,
+ "stations": "Clauss Mines,Veblen Camp"
+ },
+ {
+ "name": "Jakaliki",
+ "pos_x": 62.03125,
+ "pos_y": -61.96875,
+ "pos_z": 123,
+ "stations": "Bretnor Relay,Zhuravleva Orbital,Hall Colony"
+ },
+ {
+ "name": "Jakushu",
+ "pos_x": -25.59375,
+ "pos_y": -129.84375,
+ "pos_z": 23.625,
+ "stations": "Xuanzang Reformatory"
+ },
+ {
+ "name": "Jakuta",
+ "pos_x": 63.96875,
+ "pos_y": -120.875,
+ "pos_z": 101.40625,
+ "stations": "Mikoyan City,Malocello Works,Ludwig Struve Base"
+ },
+ {
+ "name": "Jala",
+ "pos_x": -76.5,
+ "pos_y": 30.90625,
+ "pos_z": 110.40625,
+ "stations": "Vinge Colony,Rocklynne Outpost"
+ },
+ {
+ "name": "Jalabon",
+ "pos_x": 72.3125,
+ "pos_y": -113.75,
+ "pos_z": 120.625,
+ "stations": "van de Hulst Vision,Luyten Plant,Watts Colony"
+ },
+ {
+ "name": "Jalai",
+ "pos_x": 47.03125,
+ "pos_y": -228,
+ "pos_z": 86.5625,
+ "stations": "Arkhangelsky Port,Conway Penal colony"
+ },
+ {
+ "name": "Jamab",
+ "pos_x": 139.75,
+ "pos_y": -197,
+ "pos_z": 99.28125,
+ "stations": "Curtis Point"
+ },
+ {
+ "name": "Jamalalam",
+ "pos_x": 78.46875,
+ "pos_y": -242.59375,
+ "pos_z": 19.9375,
+ "stations": "Tarter Asylum"
+ },
+ {
+ "name": "Jamanu",
+ "pos_x": 107.28125,
+ "pos_y": -13.09375,
+ "pos_z": -107.40625,
+ "stations": "Stefansson Orbital,Auer Ring,Sekelj Ring,Wafer Barracks"
+ },
+ {
+ "name": "Jamapa",
+ "pos_x": -58.5,
+ "pos_y": -28.8125,
+ "pos_z": -126.71875,
+ "stations": "Lessing Depot"
+ },
+ {
+ "name": "Jamariges",
+ "pos_x": 94.8125,
+ "pos_y": -91.03125,
+ "pos_z": 97.4375,
+ "stations": "Hay Orbital,Kidinnu Hub,Ikeya City,Sternbach Lab,Fremion Point"
+ },
+ {
+ "name": "Jamariharma",
+ "pos_x": 81.75,
+ "pos_y": 12.125,
+ "pos_z": -114.71875,
+ "stations": "Vonnegut Hub"
+ },
+ {
+ "name": "Jamat",
+ "pos_x": 77.96875,
+ "pos_y": -95.8125,
+ "pos_z": 145.40625,
+ "stations": "Henry Outpost,Molchanov's Folly"
+ },
+ {
+ "name": "Jamatia",
+ "pos_x": 14.3125,
+ "pos_y": 62.21875,
+ "pos_z": -173.53125,
+ "stations": "Tucker Station,Lorrah Port"
+ },
+ {
+ "name": "Jamaygantxe",
+ "pos_x": -52.40625,
+ "pos_y": -145.875,
+ "pos_z": 70.0625,
+ "stations": "Dingle Terminal,Fu Works"
+ },
+ {
+ "name": "Jambaraya",
+ "pos_x": -66.03125,
+ "pos_y": -184.03125,
+ "pos_z": -8.46875,
+ "stations": "Sturgeon Dock,Watt-Evans Terminal,Klein Hub,Warner Vision"
+ },
+ {
+ "name": "Jambavan",
+ "pos_x": 105.6875,
+ "pos_y": 20.8125,
+ "pos_z": 21.1875,
+ "stations": "Land Orbital,Garan Point"
+ },
+ {
+ "name": "Jambay",
+ "pos_x": -85.65625,
+ "pos_y": 142.21875,
+ "pos_z": -31.3125,
+ "stations": "Selous Reformatory,Kapp's Inheritance"
+ },
+ {
+ "name": "Jambe",
+ "pos_x": 158.03125,
+ "pos_y": -104.53125,
+ "pos_z": 120.59375,
+ "stations": "Grant Dock,Naubakht Survey"
+ },
+ {
+ "name": "Jambin",
+ "pos_x": 17.71875,
+ "pos_y": 101.15625,
+ "pos_z": 53.625,
+ "stations": "Chiang Prospect,Edison Relay,Leichhardt's Progress"
+ },
+ {
+ "name": "Jambo Dadi",
+ "pos_x": -157.5,
+ "pos_y": -31.25,
+ "pos_z": 75.625,
+ "stations": "Martin Retreat,Rolland Oasis,Vance Bastion"
+ },
+ {
+ "name": "Jambojai",
+ "pos_x": -78.59375,
+ "pos_y": -19.28125,
+ "pos_z": 101.84375,
+ "stations": "Berezovoy Mines"
+ },
+ {
+ "name": "Jambulan",
+ "pos_x": 33.59375,
+ "pos_y": -2.78125,
+ "pos_z": -158.3125,
+ "stations": "Stuart Colony,Pook Base,Francisco de Almeida Survey"
+ },
+ {
+ "name": "Jambure",
+ "pos_x": -54.84375,
+ "pos_y": 141.21875,
+ "pos_z": 11.5625,
+ "stations": "Shaara Landing,Hui Orbital"
+ },
+ {
+ "name": "Jamule",
+ "pos_x": 58.21875,
+ "pos_y": -76.5625,
+ "pos_z": -34.90625,
+ "stations": "Chernykh Terminal,Kuo City"
+ },
+ {
+ "name": "Jamuluntsu",
+ "pos_x": -72.59375,
+ "pos_y": -110.84375,
+ "pos_z": 85.34375,
+ "stations": "Hale Terminal,Dana City,Egan Observatory"
+ },
+ {
+ "name": "Jamundi",
+ "pos_x": 54.625,
+ "pos_y": -83.21875,
+ "pos_z": -112.46875,
+ "stations": "Hornoch Dock,Konscak Hub"
+ },
+ {
+ "name": "Jamurt",
+ "pos_x": -27.90625,
+ "pos_y": 25.4375,
+ "pos_z": 160,
+ "stations": "Vespucci Prospect"
+ },
+ {
+ "name": "Jamush",
+ "pos_x": 96.71875,
+ "pos_y": 58.75,
+ "pos_z": 24.46875,
+ "stations": "Saavedra Plant,Hume's Progress,Aikin Landing"
+ },
+ {
+ "name": "Jamuxa",
+ "pos_x": 34.875,
+ "pos_y": -0.6875,
+ "pos_z": 99.5,
+ "stations": "Hamilton City,Lee Dock,Cousteau Port,Samuda Orbital,Donaldson Orbital,Janifer Port"
+ },
+ {
+ "name": "Jana",
+ "pos_x": -129.09375,
+ "pos_y": 88,
+ "pos_z": 2.03125,
+ "stations": "Archimedes Point,Walker Terminal,Pawelczyk Port"
+ },
+ {
+ "name": "Jandaquiya",
+ "pos_x": 95.46875,
+ "pos_y": 19.40625,
+ "pos_z": -52.03125,
+ "stations": "Naddoddur Landing,Laird Refinery,Mitchison Penal colony"
+ },
+ {
+ "name": "Jandini",
+ "pos_x": 136.4375,
+ "pos_y": -251.625,
+ "pos_z": -1.625,
+ "stations": "Crossfield Settlement,Coande Point"
+ },
+ {
+ "name": "Jang Di",
+ "pos_x": 20.65625,
+ "pos_y": -166.71875,
+ "pos_z": 85.90625,
+ "stations": "Hevelius Dock,Mason Vision,Shea Vision,Sikorsky Orbital,Cassini Horizons,Gorgani Hub,Wilhelm von Struve Orbital,Thollon Port,Tennyson d'Eyncourt Prospect,Michael Orbital,Henry Station,Parry Hub,Pinto Prospect,Macan's Inheritance,Saha Beacon"
+ },
+ {
+ "name": "Jang O",
+ "pos_x": -71.375,
+ "pos_y": -105.65625,
+ "pos_z": -93.8125,
+ "stations": "Poleshchuk Port,Jahn Station,Sturckow City,Vittori Landing,Witt Settlement"
+ },
+ {
+ "name": "Jang Tun",
+ "pos_x": 128.25,
+ "pos_y": 77.71875,
+ "pos_z": -77.28125,
+ "stations": "Marques Station,Ordway Gateway,Salak Hub,Veblen Barracks"
+ },
+ {
+ "name": "Jangbon",
+ "pos_x": -42.40625,
+ "pos_y": -60.5,
+ "pos_z": 138.34375,
+ "stations": "Lorrah Beacon,Tiptree Mines"
+ },
+ {
+ "name": "Janggal",
+ "pos_x": 27.75,
+ "pos_y": 54.65625,
+ "pos_z": -77.59375,
+ "stations": "Wittgenstein Refinery,Glenn Base,Al-Farabi Terminal"
+ },
+ {
+ "name": "Jangkarada",
+ "pos_x": -113.5625,
+ "pos_y": -23.09375,
+ "pos_z": -43.53125,
+ "stations": "Tapinas Plant,Yaping Colony,Wingrove Mine,Meikle Platform"
+ },
+ {
+ "name": "Jangman",
+ "pos_x": -142.375,
+ "pos_y": -7.625,
+ "pos_z": -66.375,
+ "stations": "Killough Legacy,Malzberg Reach,Tiedemann's Inheritance"
+ },
+ {
+ "name": "Jangore",
+ "pos_x": -39.1875,
+ "pos_y": -3.1875,
+ "pos_z": -86,
+ "stations": "Meikle Survey,Guest Port,Ings Landing"
+ },
+ {
+ "name": "Jangu",
+ "pos_x": 116.9375,
+ "pos_y": 87.65625,
+ "pos_z": -16.75,
+ "stations": "Cleve Terminal"
+ },
+ {
+ "name": "Janguti",
+ "pos_x": 89,
+ "pos_y": -123.875,
+ "pos_z": 131.4375,
+ "stations": "Impey Orbital,Skolem Town"
+ },
+ {
+ "name": "Janja",
+ "pos_x": 153.8125,
+ "pos_y": -79.34375,
+ "pos_z": 17.59375,
+ "stations": "Koishikawa Port,Bolger Silo,Allen Dock,Kawanishi Horizons"
+ },
+ {
+ "name": "Janjiwa",
+ "pos_x": 56.03125,
+ "pos_y": 67.40625,
+ "pos_z": 121.8125,
+ "stations": "Bunch Survey,White Station,Poincare Prospect,McMullen Port,Gallun Bastion"
+ },
+ {
+ "name": "Jannonandji",
+ "pos_x": 68.34375,
+ "pos_y": -42.375,
+ "pos_z": 119.09375,
+ "stations": "Manning Dock,Wagner Arsenal,Webb Settlement"
+ },
+ {
+ "name": "Jannos",
+ "pos_x": -96.6875,
+ "pos_y": -84.5625,
+ "pos_z": 89.96875,
+ "stations": "Hadamard Installation,Moresby Vision,Cantor Terminal"
+ },
+ {
+ "name": "Jaoi",
+ "pos_x": -118.09375,
+ "pos_y": 54.65625,
+ "pos_z": -72.4375,
+ "stations": "Soto Dock,Weyl Orbital,Thompson Terminal"
+ },
+ {
+ "name": "Jaradharre",
+ "pos_x": 39.53125,
+ "pos_y": 21.28125,
+ "pos_z": 56.625,
+ "stations": "Gohar Station,Cernan Dock,Beekman Point,Bunnell Point,Szebehely Hub"
+ },
+ {
+ "name": "Jarakati",
+ "pos_x": 157.625,
+ "pos_y": -62.40625,
+ "pos_z": 74.34375,
+ "stations": "Walotsky Mines,Wirtanen Survey,Shawl Base"
+ },
+ {
+ "name": "Jaralland",
+ "pos_x": -7.3125,
+ "pos_y": -76.53125,
+ "pos_z": -65.4375,
+ "stations": "Lloyd Wright Arsenal,Efremov Prospect,Petaja Settlement"
+ },
+ {
+ "name": "Jardama",
+ "pos_x": 55.78125,
+ "pos_y": -136.96875,
+ "pos_z": 86.09375,
+ "stations": "Barbosa Bastion,Ulloa's Claim"
+ },
+ {
+ "name": "Jardha",
+ "pos_x": 40.375,
+ "pos_y": 79.21875,
+ "pos_z": -119.6875,
+ "stations": "Brunel Dock,Grover Orbital,Kovalyonok Terminal"
+ },
+ {
+ "name": "Jardonnere",
+ "pos_x": 133.9375,
+ "pos_y": 59.8125,
+ "pos_z": 106.78125,
+ "stations": "Bloch Survey,Kovalevskaya Prospect,Kube-McDowell Prospect,Cook Camp"
+ },
+ {
+ "name": "Jardoviceni",
+ "pos_x": -102.40625,
+ "pos_y": 66.5,
+ "pos_z": -144.53125,
+ "stations": "Rond d'Alembert Hub,Lanier Colony,Levi-Civita Hub,Andersson Point,Nagata Relay"
+ },
+ {
+ "name": "Jardovici",
+ "pos_x": -7.78125,
+ "pos_y": -86.53125,
+ "pos_z": -9.3125,
+ "stations": "Shepherd Orbital,Guerrero Prospect,Resnick Silo"
+ },
+ {
+ "name": "Jarics",
+ "pos_x": -118.75,
+ "pos_y": -80.46875,
+ "pos_z": -82.34375,
+ "stations": "Hale Landing,Hopkins Port"
+ },
+ {
+ "name": "Jarildekald",
+ "pos_x": -164.9375,
+ "pos_y": 23.21875,
+ "pos_z": -62.875,
+ "stations": "Elvstrom Platform,Carlisle Landing,Reed Stop"
+ },
+ {
+ "name": "Jaring",
+ "pos_x": -14.71875,
+ "pos_y": 75.96875,
+ "pos_z": 136.375,
+ "stations": "Budarin Depot"
+ },
+ {
+ "name": "Jarnae",
+ "pos_x": -29.15625,
+ "pos_y": -39,
+ "pos_z": 33.90625,
+ "stations": "Powell Platform,Kagan Installation,Evangelisti Holdings"
+ },
+ {
+ "name": "Jarni",
+ "pos_x": -56.96875,
+ "pos_y": -67.78125,
+ "pos_z": -12.4375,
+ "stations": "McAllaster's Claim"
+ },
+ {
+ "name": "Jarnsaxa",
+ "pos_x": 142.1875,
+ "pos_y": -65.125,
+ "pos_z": -66.09375,
+ "stations": "Thorne Sanctuary"
+ },
+ {
+ "name": "Jarnuekenk",
+ "pos_x": -18,
+ "pos_y": -13.53125,
+ "pos_z": 103.0625,
+ "stations": "Popper Orbital,Brillant Vision,Nikitin Station,Stephenson Gateway"
+ },
+ {
+ "name": "Jarnun",
+ "pos_x": -8.375,
+ "pos_y": -170.28125,
+ "pos_z": -37.875,
+ "stations": "Kowal Settlement,Rabinowitz Dock,Beliaev Silo"
+ },
+ {
+ "name": "Jaroahy",
+ "pos_x": 100.5625,
+ "pos_y": 19.21875,
+ "pos_z": 78.375,
+ "stations": "Chamitoff Horizons,Tereshkova Terminal,Fremion Bastion,Celebi Lab"
+ },
+ {
+ "name": "Jaroere",
+ "pos_x": -18.5625,
+ "pos_y": -22.25,
+ "pos_z": 96.46875,
+ "stations": "Moore Base,Shirley Hub,Rothman Depot"
+ },
+ {
+ "name": "Jarog",
+ "pos_x": -88.3125,
+ "pos_y": 43.625,
+ "pos_z": -148.28125,
+ "stations": "Pelliot Terminal,Stokes Prospect"
+ },
+ {
+ "name": "Jarogee",
+ "pos_x": -2.0625,
+ "pos_y": -143.46875,
+ "pos_z": 45.5625,
+ "stations": "Newcomb Orbital,Dawes Hub,Fabian Port,Spurzem Terminal,Mooz Terminal,Wafer's Inheritance,Ritchey Vision,Watts Holdings"
+ },
+ {
+ "name": "Jaroji",
+ "pos_x": -109.40625,
+ "pos_y": 99.75,
+ "pos_z": -29.3125,
+ "stations": "Auld Depot,Shaver's Inheritance"
+ },
+ {
+ "name": "Jarojinanh",
+ "pos_x": -24.5625,
+ "pos_y": -168.53125,
+ "pos_z": 109.625,
+ "stations": "Daqing Relay,Osterbrock Dock,Carr Bastion"
+ },
+ {
+ "name": "Jarorotri",
+ "pos_x": -89.0625,
+ "pos_y": -11.6875,
+ "pos_z": -131.03125,
+ "stations": "Yaping Gateway,Rzeppa Enterprise,Ponce de Leon Enterprise,Bramah Terminal"
+ },
+ {
+ "name": "Jaroua",
+ "pos_x": 157.53125,
+ "pos_y": -110.53125,
+ "pos_z": 28.25,
+ "stations": "McCool City,Schwarzschild Port,Asher Landing,Bisson Hub,Hayashi Enterprise,Sabine Laboratory"
+ },
+ {
+ "name": "Jarovit",
+ "pos_x": 116.5625,
+ "pos_y": -118.125,
+ "pos_z": -51.3125,
+ "stations": "Canty Landing,Hudson Survey"
+ },
+ {
+ "name": "Jarowair",
+ "pos_x": 9.96875,
+ "pos_y": 83.53125,
+ "pos_z": -74.53125,
+ "stations": "Julian Hub,Lenthall Enterprise,McQuay Mines,Gloss Survey,Zetford Enterprise,Hunt Beacon"
+ },
+ {
+ "name": "Jasinatlehi",
+ "pos_x": -163.09375,
+ "pos_y": 60,
+ "pos_z": 60.71875,
+ "stations": "Cramer Prospect"
+ },
+ {
+ "name": "Jasira",
+ "pos_x": 169.78125,
+ "pos_y": -159.3125,
+ "pos_z": -2.75,
+ "stations": "Wright Dock,Gaspar de Lemos Landing"
+ },
+ {
+ "name": "Jasiri",
+ "pos_x": -125.28125,
+ "pos_y": 48.71875,
+ "pos_z": 33.84375,
+ "stations": "Stewart Terminal,Curbeam Landing,Vardeman's Inheritance"
+ },
+ {
+ "name": "Jatana",
+ "pos_x": -99.9375,
+ "pos_y": 56.3125,
+ "pos_z": -142.75,
+ "stations": "Sheffield Dock,Hausdorff Settlement,Stephens Lab"
+ },
+ {
+ "name": "Jatanthet",
+ "pos_x": 57.09375,
+ "pos_y": -44.875,
+ "pos_z": -116.90625,
+ "stations": "Thirsk Point,Stephenson Works"
+ },
+ {
+ "name": "Jatar",
+ "pos_x": 103.5,
+ "pos_y": -100.21875,
+ "pos_z": 133.21875,
+ "stations": "Ghez Dock,Alfven Dock,Wilhelm von Struve Terminal,Archer's Progress"
+ },
+ {
+ "name": "Jataya",
+ "pos_x": -36.40625,
+ "pos_y": -67.96875,
+ "pos_z": -152.71875,
+ "stations": "England Hub,Godwin Prospect,Metcalf Dock,Gibson Settlement,Sarafanov Survey"
+ },
+ {
+ "name": "Jath",
+ "pos_x": 56.71875,
+ "pos_y": 58.6875,
+ "pos_z": 98.4375,
+ "stations": "Ricci Port,Nicholson Landing,Kuttner Relay,Crown Terminal"
+ },
+ {
+ "name": "Jathaikana",
+ "pos_x": -10.6875,
+ "pos_y": 28.03125,
+ "pos_z": -148.53125,
+ "stations": "Ham Port,Skvortsov Gateway,Acton Orbital,Ellern Landing"
+ },
+ {
+ "name": "Jathalyod",
+ "pos_x": 60.6875,
+ "pos_y": -184.28125,
+ "pos_z": 125.1875,
+ "stations": "Rizvi Vision,Kurtz Port,Celsius Relay"
+ },
+ {
+ "name": "Jathatin",
+ "pos_x": 157.65625,
+ "pos_y": 52.65625,
+ "pos_z": -55.09375,
+ "stations": "Smith Dock,Ramsbottom Colony"
+ },
+ {
+ "name": "Jaudugayo",
+ "pos_x": 49,
+ "pos_y": 62.03125,
+ "pos_z": -45.84375,
+ "stations": "Rand Colony"
+ },
+ {
+ "name": "Jaunera",
+ "pos_x": 56.34375,
+ "pos_y": 56.96875,
+ "pos_z": 54.84375,
+ "stations": "Fraley Laboratory,Engle Station"
+ },
+ {
+ "name": "Jaung",
+ "pos_x": 69.1875,
+ "pos_y": -30.90625,
+ "pos_z": 39.96875,
+ "stations": "Mattingly Hangar"
+ },
+ {
+ "name": "Jauri He",
+ "pos_x": 159.9375,
+ "pos_y": -136.40625,
+ "pos_z": 128.375,
+ "stations": "Beer Holdings,Friedman Oasis,White's Folly,Davis' Inheritance"
+ },
+ {
+ "name": "Jaurinani",
+ "pos_x": -19.53125,
+ "pos_y": -36.96875,
+ "pos_z": 134.84375,
+ "stations": "Ellison Co-operative,Morris Depot,Langsdorff Installation"
+ },
+ {
+ "name": "Jawola",
+ "pos_x": 61.96875,
+ "pos_y": 48.6875,
+ "pos_z": 112.5,
+ "stations": "Sutcliffe Platform"
+ },
+ {
+ "name": "Jawul",
+ "pos_x": -140.34375,
+ "pos_y": -72.34375,
+ "pos_z": -4.09375,
+ "stations": "Rushd City,Anders Installation,de Andrade Survey,Savinykh City,McKay Orbital"
+ },
+ {
+ "name": "Jawuru",
+ "pos_x": -15.1875,
+ "pos_y": 62.28125,
+ "pos_z": 128.4375,
+ "stations": "Jenkinson Freeport,Guerrero Prospect,Wright Point,Blaylock Survey"
+ },
+ {
+ "name": "Jeid",
+ "pos_x": 83.125,
+ "pos_y": 71.375,
+ "pos_z": -82.8125,
+ "stations": "Nagel Holdings"
+ },
+ {
+ "name": "Jeidenannsa",
+ "pos_x": -103.34375,
+ "pos_y": -89.125,
+ "pos_z": -120.5625,
+ "stations": "Sekowski Legacy,Brooks Prospect,Stackpole Plant"
+ },
+ {
+ "name": "Jeidubia",
+ "pos_x": 6.46875,
+ "pos_y": -98.53125,
+ "pos_z": 99.34375,
+ "stations": "Rechtin Dock,Cheranovsky's Folly,Ohain Mines"
+ },
+ {
+ "name": "Jeirom",
+ "pos_x": 42.6875,
+ "pos_y": -152.78125,
+ "pos_z": -79.4375,
+ "stations": "Bode Port,Verrier Legacy,Stabenow Relay,Chorel Silo"
+ },
+ {
+ "name": "Jeiromen",
+ "pos_x": 56.53125,
+ "pos_y": -132.03125,
+ "pos_z": 170.875,
+ "stations": "Longomontanus Relay,Schroeder Installation"
+ },
+ {
+ "name": "Jeirretes",
+ "pos_x": 113,
+ "pos_y": -119.5625,
+ "pos_z": 22.125,
+ "stations": "Schmidt Port,Evans Terminal,Beer Station"
+ },
+ {
+ "name": "Jeirskoku",
+ "pos_x": -121.46875,
+ "pos_y": 37.25,
+ "pos_z": 0.28125,
+ "stations": "Tanner Hub,Steakley Hub,Ellern Hub,Vancouver Arena"
+ },
+ {
+ "name": "Jeit",
+ "pos_x": 169.90625,
+ "pos_y": -19.4375,
+ "pos_z": -21.40625,
+ "stations": "Kippax Landing,Weiss Mine"
+ },
+ {
+ "name": "Jeiteswar",
+ "pos_x": -56.25,
+ "pos_y": -62.15625,
+ "pos_z": 79.9375,
+ "stations": "Sarmiento de Gamboa Settlement"
+ },
+ {
+ "name": "Jeitjali",
+ "pos_x": 61.9375,
+ "pos_y": 5.5625,
+ "pos_z": -106,
+ "stations": "Kiernan Refinery"
+ },
+ {
+ "name": "Jeitsegezi",
+ "pos_x": 72.40625,
+ "pos_y": -201.6875,
+ "pos_z": -40.28125,
+ "stations": "Griffin Bastion,Firsoff Landing,Hogan Installation,Battani Landing"
+ },
+ {
+ "name": "Jeitsing",
+ "pos_x": 98.1875,
+ "pos_y": -130.03125,
+ "pos_z": -49.375,
+ "stations": "Shinjo Ring,Gehry Keep"
+ },
+ {
+ "name": "Jeljendii",
+ "pos_x": -121.3125,
+ "pos_y": 53.8125,
+ "pos_z": 118.65625,
+ "stations": "Tanner Dock,Huygens Mines,Ramsbottom Port"
+ },
+ {
+ "name": "Jellyfish Sector FB-X c1-5",
+ "pos_x": 788.40625,
+ "pos_y": 255.46875,
+ "pos_z": -4946.4375,
+ "stations": "Beta Site"
+ },
+ {
+ "name": "Jemede",
+ "pos_x": 27.15625,
+ "pos_y": -171.34375,
+ "pos_z": -6.8125,
+ "stations": "Dhawan Platform,Kohoutek Mines"
+ },
+ {
+ "name": "Jemehuenges",
+ "pos_x": 115.375,
+ "pos_y": 110.78125,
+ "pos_z": -80.46875,
+ "stations": "Bailey Dock,Pytheas Dock,Alexeyev Platform,Saavedra Silo"
+ },
+ {
+ "name": "Jemen",
+ "pos_x": 49.53125,
+ "pos_y": -152.0625,
+ "pos_z": -33.0625,
+ "stations": "Kushida Terminal,Roth Terminal,Tank Landing,Naboth Terminal"
+ },
+ {
+ "name": "Jementi",
+ "pos_x": -48.625,
+ "pos_y": -135.34375,
+ "pos_z": -91.25,
+ "stations": "Donaldson Mine,Weber's Claim,Janes' Inheritance,Pinto's Inheritance"
+ },
+ {
+ "name": "Jementunde",
+ "pos_x": -92.9375,
+ "pos_y": -102.78125,
+ "pos_z": 92.15625,
+ "stations": "Johnson Mine"
+ },
+ {
+ "name": "Jemetani",
+ "pos_x": -41.625,
+ "pos_y": -94.9375,
+ "pos_z": -18.90625,
+ "stations": "Henry Plant,Lovelace Hangar,Shonin Depot"
+ },
+ {
+ "name": "Jen Elabog",
+ "pos_x": -44.15625,
+ "pos_y": -29.9375,
+ "pos_z": 36.125,
+ "stations": "Sarich Port,Shepard Port,Bresnik Orbital,Sarrantonio Point"
+ },
+ {
+ "name": "Jen Kunguru",
+ "pos_x": 158.96875,
+ "pos_y": -76.21875,
+ "pos_z": 71.34375,
+ "stations": "Florine Terminal"
+ },
+ {
+ "name": "Jen Lung",
+ "pos_x": 87.34375,
+ "pos_y": -164.40625,
+ "pos_z": 84.5625,
+ "stations": "Kirkwood Station,Very Port"
+ },
+ {
+ "name": "Jen Nik",
+ "pos_x": -20.9375,
+ "pos_y": -43.34375,
+ "pos_z": 145.625,
+ "stations": "Bester Terminal"
+ },
+ {
+ "name": "Jen Tienses",
+ "pos_x": 85.96875,
+ "pos_y": -44.3125,
+ "pos_z": 117.875,
+ "stations": "Sturgeon's Inheritance,Perry's Inheritance,Matthaus Olbers Port,Truly Port"
+ },
+ {
+ "name": "Jeng",
+ "pos_x": -75.90625,
+ "pos_y": 42.53125,
+ "pos_z": -90.75,
+ "stations": "Rothman Plant,Ore's Pride"
+ },
+ {
+ "name": "Jenu",
+ "pos_x": -139.4375,
+ "pos_y": 71.625,
+ "pos_z": 36.09375,
+ "stations": "Konscak Station,Morrison Hub,Maire Settlement,Taine Terminal,McHugh Base"
+ },
+ {
+ "name": "Jera",
+ "pos_x": 30.9375,
+ "pos_y": -83.21875,
+ "pos_z": 46.125,
+ "stations": "Asire Dock,Coney Port,Moore Dock,Mitchell Orbital,Rechtin Gateway,Lee City"
+ },
+ {
+ "name": "Jetenomeri",
+ "pos_x": 97.78125,
+ "pos_y": -191.5,
+ "pos_z": 5.8125,
+ "stations": "Brin Refinery,Heinkel Mines,Bradfield Station"
+ },
+ {
+ "name": "Jeterait",
+ "pos_x": 64.5625,
+ "pos_y": -85.125,
+ "pos_z": 42.1875,
+ "stations": "Barnard Port,Skjellerup Terminal,Barnwell Station,Helin Terminal,McIntosh Terminal,Rayhan al-Biruni Vision,Kaluta's Folly,Sabine Orbital,Seyfert Dock,Isaev Settlement,Derekas Reach,Malaspina Depot,Vardeman Settlement,Dean Base"
+ },
+ {
+ "name": "Jetes",
+ "pos_x": -141.21875,
+ "pos_y": 16.28125,
+ "pos_z": -59.3125,
+ "stations": "Cook Prospect,Sheffield Settlement,Goulart Installation,Taylor Point"
+ },
+ {
+ "name": "Jeti",
+ "pos_x": -122.1875,
+ "pos_y": 24.59375,
+ "pos_z": 36.84375,
+ "stations": "Rasmussen Port,Plante Station,MacCurdy City,Clark Gateway,Wylie Terminal"
+ },
+ {
+ "name": "Jeticans",
+ "pos_x": 28.90625,
+ "pos_y": 11.0625,
+ "pos_z": -110.125,
+ "stations": "Helms Depot,Puleston Vision"
+ },
+ {
+ "name": "Jian",
+ "pos_x": -100.6875,
+ "pos_y": -1,
+ "pos_z": 113,
+ "stations": "Warner Landing,Staden Dock,Amnuel Works"
+ },
+ {
+ "name": "Jian Yu",
+ "pos_x": -95.6875,
+ "pos_y": 40.75,
+ "pos_z": 69.8125,
+ "stations": "Saunders Orbital,Shaara Beacon,Neumann Silo,Hugh Prospect"
+ },
+ {
+ "name": "Jiang",
+ "pos_x": 73.625,
+ "pos_y": -162.75,
+ "pos_z": 111.25,
+ "stations": "Shoemaker Station,Donati Ring,Sato Enterprise,Palitzsch Relay,Lethem Camp"
+ },
+ {
+ "name": "Jibio",
+ "pos_x": 142.46875,
+ "pos_y": -60,
+ "pos_z": -12.34375,
+ "stations": "Gerrold Dock,Binder Prospect,Bisson Prospect,Speke Reach"
+ },
+ {
+ "name": "Jibitoq",
+ "pos_x": 13.09375,
+ "pos_y": -68.875,
+ "pos_z": -30.71875,
+ "stations": "Mach Dock,Wellman Gateway,Haberlandt Dock,Valdes Plant,Scheutz Holdings"
+ },
+ {
+ "name": "Jicarex",
+ "pos_x": -95.25,
+ "pos_y": -34.21875,
+ "pos_z": 53.46875,
+ "stations": "Lee Dock,Wingrove Arsenal,Cook Prospect"
+ },
+ {
+ "name": "Jicasses",
+ "pos_x": 132.09375,
+ "pos_y": -251.5625,
+ "pos_z": -42.9375,
+ "stations": "McKee Terminal"
+ },
+ {
+ "name": "Jicaura",
+ "pos_x": -127.8125,
+ "pos_y": 68.3125,
+ "pos_z": 12.90625,
+ "stations": "Onizuka Settlement,Stebler Settlement,Laumer Point"
+ },
+ {
+ "name": "Jieguaje",
+ "pos_x": -93.46875,
+ "pos_y": 32.1875,
+ "pos_z": 1.9375,
+ "stations": "Kettle Horizons,Appel Observatory"
+ },
+ {
+ "name": "Jikoku",
+ "pos_x": 150.3125,
+ "pos_y": -70.125,
+ "pos_z": 30.5,
+ "stations": "Mason Platform"
+ },
+ {
+ "name": "Jilnytis",
+ "pos_x": 83.03125,
+ "pos_y": -20.625,
+ "pos_z": 19.96875,
+ "stations": "Mikoyan Ring,Rasch Relay,Weber Oasis"
+ },
+ {
+ "name": "Jimai",
+ "pos_x": 117.03125,
+ "pos_y": -162.28125,
+ "pos_z": 39.96875,
+ "stations": "Mies van der Rohe Colony,Mead Settlement"
+ },
+ {
+ "name": "Jiman",
+ "pos_x": -26.625,
+ "pos_y": 100.625,
+ "pos_z": -15.46875,
+ "stations": "Kneale Hub,Karlsefni Survey,Paulmier de Gonneville's Claim"
+ },
+ {
+ "name": "Jimara",
+ "pos_x": 107.90625,
+ "pos_y": -44.5625,
+ "pos_z": 30.25,
+ "stations": "Spurzem Terminal,Chernykh Hub,Hoffleit Port,Matthews Station,Harding Station,Huggins Station,Ptack Terminal"
+ },
+ {
+ "name": "Jimatch",
+ "pos_x": 145.8125,
+ "pos_y": -178.90625,
+ "pos_z": 73.4375,
+ "stations": "August von Steinheil Base"
+ },
+ {
+ "name": "Jimavi",
+ "pos_x": 79.75,
+ "pos_y": -85.8125,
+ "pos_z": 14.3125,
+ "stations": "Naylor Installation,Hansen Platform"
+ },
+ {
+ "name": "Jin Sho Bao",
+ "pos_x": -117.03125,
+ "pos_y": 107.53125,
+ "pos_z": -11.6875,
+ "stations": "Russell Horizons,Henderson Horizons,Farkas Installation"
+ },
+ {
+ "name": "Jing",
+ "pos_x": 62.65625,
+ "pos_y": 62.75,
+ "pos_z": 40.90625,
+ "stations": "Onizuka Vision,Lavoisier Dock"
+ },
+ {
+ "name": "Jinga",
+ "pos_x": 130.5,
+ "pos_y": -20.40625,
+ "pos_z": -18.4375,
+ "stations": "Haxel's Folly,Popov Colony,Nakaya Settlement"
+ },
+ {
+ "name": "Jingaana",
+ "pos_x": 36.28125,
+ "pos_y": 165.28125,
+ "pos_z": 40.3125,
+ "stations": "Horch Retreat"
+ },
+ {
+ "name": "Jinganago",
+ "pos_x": -30,
+ "pos_y": -116.90625,
+ "pos_z": 105.59375,
+ "stations": "Seares Port,Herschel Landing"
+ },
+ {
+ "name": "Jingones",
+ "pos_x": -126,
+ "pos_y": 38.375,
+ "pos_z": 70.375,
+ "stations": "Lanchester Outpost,Carpenter Point"
+ },
+ {
+ "name": "Jingpo",
+ "pos_x": 88.90625,
+ "pos_y": 67.40625,
+ "pos_z": 19.0625,
+ "stations": "Alcala Colony,Hire Prospect,Kagan Refinery"
+ },
+ {
+ "name": "Jinicoch",
+ "pos_x": -21.71875,
+ "pos_y": 63.46875,
+ "pos_z": 39.15625,
+ "stations": "Coulomb City,Culbertson Terminal,Gooch Enterprise,Feustel Terminal,Tryggvason Station,Great Refinery,Mukai Hub,Belyayev Orbital,Barreiros Vision,Shriver Hub,Aleksandrov Dock,Broglie Hub"
+ },
+ {
+ "name": "Jinighu",
+ "pos_x": -65.25,
+ "pos_y": -80.75,
+ "pos_z": -122.34375,
+ "stations": "Kozlov Terminal,Williamson Platform,Arthur's Progress"
+ },
+ {
+ "name": "Jinoharis",
+ "pos_x": 74.09375,
+ "pos_y": 7.09375,
+ "pos_z": 29.625,
+ "stations": "Pogue Point,Humphreys Vision,Korzun Platform"
+ },
+ {
+ "name": "Jirani",
+ "pos_x": -155.5625,
+ "pos_y": 62.03125,
+ "pos_z": 32.25,
+ "stations": "Abel Platform,Lewis Orbital,Compton Port,Obruchev Vision"
+ },
+ {
+ "name": "Jirara",
+ "pos_x": 71.40625,
+ "pos_y": -173.25,
+ "pos_z": 150.34375,
+ "stations": "Bohr Horizons,Nahavandi Gateway,Eanes Holdings,Kapp Enterprise"
+ },
+ {
+ "name": "Jita",
+ "pos_x": 63.8125,
+ "pos_y": -89.1875,
+ "pos_z": -74.875,
+ "stations": "Shatner Enterprise,Cleve Survey,Matthaus Olbers Dock,Arai Platform,Grant Station"
+ },
+ {
+ "name": "Jita Ten",
+ "pos_x": 26.28125,
+ "pos_y": 11.6875,
+ "pos_z": -74.5,
+ "stations": "Balandin Enterprise,Yolen Settlement,Barry Gateway"
+ },
+ {
+ "name": "Jitabed",
+ "pos_x": -19.75,
+ "pos_y": -0.78125,
+ "pos_z": -115.84375,
+ "stations": "Meitner Gateway,Weber Station,Lind Station,Beliaev Landing"
+ },
+ {
+ "name": "Jitabos",
+ "pos_x": -119.65625,
+ "pos_y": 83.15625,
+ "pos_z": -8.21875,
+ "stations": "Payson Terminal,Erdos Port,Finney Ring,Gregory's Folly,Andersson City,Shaver Point"
+ },
+ {
+ "name": "Jitani",
+ "pos_x": -157.53125,
+ "pos_y": -6.15625,
+ "pos_z": 41.25,
+ "stations": "Padalka Relay,Baker Dock,Slade Dock,Peral Enterprise"
+ },
+ {
+ "name": "Jitar",
+ "pos_x": -104.4375,
+ "pos_y": -34.96875,
+ "pos_z": 17.71875,
+ "stations": "Samuda Installation,Robinson Installation,Dampier Depot"
+ },
+ {
+ "name": "Jiuyou",
+ "pos_x": 24,
+ "pos_y": -4.09375,
+ "pos_z": 38.15625,
+ "stations": "Poncelet Survey,Keyes Refinery,Hiraga Arsenal,Birmingham Freeport"
+ },
+ {
+ "name": "Jivaraikpoi",
+ "pos_x": -80.5625,
+ "pos_y": -77.9375,
+ "pos_z": 47.25,
+ "stations": "Boole Beacon,Ray Terminal"
+ },
+ {
+ "name": "Jodrell",
+ "pos_x": 34.875,
+ "pos_y": 43.4375,
+ "pos_z": 64.0625,
+ "stations": "Jodrell Bank,Duxford Station"
+ },
+ {
+ "name": "Jofnour",
+ "pos_x": -20.40625,
+ "pos_y": -91.5,
+ "pos_z": 35.78125,
+ "stations": "Dunn City,Sabine Point,Durrance Hub,Margulis Terminal,Baird Orbital"
+ },
+ {
+ "name": "Joh",
+ "pos_x": 162.03125,
+ "pos_y": -16,
+ "pos_z": 30.53125,
+ "stations": "Thorne Platform,Velho's Inheritance,Crown Port"
+ },
+ {
+ "name": "Jok Islese",
+ "pos_x": 70.15625,
+ "pos_y": 51.5625,
+ "pos_z": 9.5,
+ "stations": "Fox Orbital,Lukyanenko Port,Hamilton Base"
+ },
+ {
+ "name": "Jok Isuani",
+ "pos_x": 17.5,
+ "pos_y": 57.78125,
+ "pos_z": 98.3125,
+ "stations": "Chang-Diaz Orbital,Illy Hub,Cassidy Port,Macedo Installation"
+ },
+ {
+ "name": "Jok Odudu",
+ "pos_x": 59.21875,
+ "pos_y": -132.4375,
+ "pos_z": -48.65625,
+ "stations": "August von Steinheil Vision,Samos Port,Ikeya Vision"
+ },
+ {
+ "name": "Joku",
+ "pos_x": 76.875,
+ "pos_y": -238,
+ "pos_z": 64.71875,
+ "stations": "Takahashi Station,Bok Oasis"
+ },
+ {
+ "name": "Jokudiri",
+ "pos_x": 150.34375,
+ "pos_y": -188.65625,
+ "pos_z": 46.84375,
+ "stations": "Krigstein Prospect,Vonarburg Base,Asami Landing,Jekhowsky Vision,Ban Installation"
+ },
+ {
+ "name": "Jolagro",
+ "pos_x": 14.75,
+ "pos_y": -100.625,
+ "pos_z": -161.1875,
+ "stations": "Gentle Survey,Kneale Escape"
+ },
+ {
+ "name": "Jolanji",
+ "pos_x": 85.3125,
+ "pos_y": 128.71875,
+ "pos_z": -54.5625,
+ "stations": "Thirsk Relay,Ampere Arena"
+ },
+ {
+ "name": "Jolapa",
+ "pos_x": 100.1875,
+ "pos_y": -41.34375,
+ "pos_z": -78,
+ "stations": "Crouch Stop,Bobko Mines"
+ },
+ {
+ "name": "Jonai",
+ "pos_x": -99.8125,
+ "pos_y": 18.09375,
+ "pos_z": 86.40625,
+ "stations": "Pribylov Port,Kovalevskaya Holdings,Anvil Hub,Silves Keep"
+ },
+ {
+ "name": "Jongo",
+ "pos_x": 140.46875,
+ "pos_y": -160.1875,
+ "pos_z": -41.9375,
+ "stations": "Robert Relay"
+ },
+ {
+ "name": "Jongsaki",
+ "pos_x": 30.75,
+ "pos_y": 94.9375,
+ "pos_z": 22.5625,
+ "stations": "Wrangell Horizons,Thompson Enterprise,Bartlett Installation"
+ },
+ {
+ "name": "Jongsang",
+ "pos_x": 46.5,
+ "pos_y": 12.96875,
+ "pos_z": 102.53125,
+ "stations": "Baffin Base,Whitson Vision,Doi Terminal"
+ },
+ {
+ "name": "Jormbu",
+ "pos_x": -112.03125,
+ "pos_y": 65.15625,
+ "pos_z": 58.75,
+ "stations": "Mendez Terminal,Erdos Hub,Gotlieb Lab"
+ },
+ {
+ "name": "Jormbuti",
+ "pos_x": -10.1875,
+ "pos_y": -14.0625,
+ "pos_z": 112.6875,
+ "stations": "Ocampo Legacy,Fozard Refinery"
+ },
+ {
+ "name": "Jormor",
+ "pos_x": -140.90625,
+ "pos_y": -17.8125,
+ "pos_z": 18.0625,
+ "stations": "Cardano Port,Archambault Gateway,Laue Terminal,Dashiell's Pride,Newcomen Refinery"
+ },
+ {
+ "name": "Jormungand",
+ "pos_x": 32.125,
+ "pos_y": -164.625,
+ "pos_z": -101.03125,
+ "stations": "Ahnert-Rohlfs Gateway,Boss Terminal,Challis Terminal,McKie Relay,Maler Installation"
+ },
+ {
+ "name": "Joro",
+ "pos_x": 15.375,
+ "pos_y": 30.03125,
+ "pos_z": -63.34375,
+ "stations": "White Silo,Brunel Station,Maclaurin Prospect"
+ },
+ {
+ "name": "Jotnar",
+ "pos_x": -54.625,
+ "pos_y": 24.3125,
+ "pos_z": -86.21875,
+ "stations": "Crown Station"
+ },
+ {
+ "name": "Jotun",
+ "pos_x": -11.03125,
+ "pos_y": -79.21875,
+ "pos_z": -92.3125,
+ "stations": "Icelock"
+ },
+ {
+ "name": "Jotunheim",
+ "pos_x": 66.03125,
+ "pos_y": -110.875,
+ "pos_z": 52.6875,
+ "stations": "Big Harry's Monkey Hangout,Laird Enterprise,Henson Station,Dufay Barracks"
+ },
+ {
+ "name": "Jou Tung",
+ "pos_x": 138.84375,
+ "pos_y": -21.96875,
+ "pos_z": 76.625,
+ "stations": "Padalka Station,Ricci Prospect,Avogadro Dock,Chilton Ring,Schiltberger Plant"
+ },
+ {
+ "name": "Jou Wang",
+ "pos_x": 46.96875,
+ "pos_y": 115.09375,
+ "pos_z": -36,
+ "stations": "Hartog Relay,Cartier's Inheritance"
+ },
+ {
+ "name": "Ju Shakhe",
+ "pos_x": 123.5,
+ "pos_y": 8.46875,
+ "pos_z": 24.03125,
+ "stations": "Klimuk Ring,Kooi Hub,Melvin Base"
+ },
+ {
+ "name": "Ju Sheng",
+ "pos_x": -1.28125,
+ "pos_y": 125.4375,
+ "pos_z": -58.40625,
+ "stations": "Antonelli Works"
+ },
+ {
+ "name": "Ju Shiva",
+ "pos_x": 143.78125,
+ "pos_y": -56.1875,
+ "pos_z": 37.125,
+ "stations": "Newcomb Station"
+ },
+ {
+ "name": "Ju Shosi",
+ "pos_x": 132.5,
+ "pos_y": -110.8125,
+ "pos_z": 79.40625,
+ "stations": "Davies Dock,De Lay Point,Samuda Prospect"
+ },
+ {
+ "name": "Ju Shou Yax",
+ "pos_x": 59.03125,
+ "pos_y": 17.4375,
+ "pos_z": 69.5625,
+ "stations": "Laumer Installation,Broglie Outpost,Fontana Mines"
+ },
+ {
+ "name": "Juan",
+ "pos_x": -85,
+ "pos_y": -6.0625,
+ "pos_z": -24.46875,
+ "stations": "Lyell Hub,Peirce Silo,Fernao do Po Camp"
+ },
+ {
+ "name": "Juato",
+ "pos_x": 123.28125,
+ "pos_y": -177.34375,
+ "pos_z": 132.3125,
+ "stations": "Delporte Point,Hoffmeister's Progress"
+ },
+ {
+ "name": "Judumlia",
+ "pos_x": 8.78125,
+ "pos_y": -136.40625,
+ "pos_z": -89.0625,
+ "stations": "Rawat City,Knight Prospect,Parry Station,O'Leary City,Wilson Installation"
+ },
+ {
+ "name": "Juduni",
+ "pos_x": -96.6875,
+ "pos_y": 143.15625,
+ "pos_z": -3.9375,
+ "stations": "Thompson Ring,Macarthur Mines,Rontgen City,Pelt Installation,Clute Depot"
+ },
+ {
+ "name": "Juipedi",
+ "pos_x": 90.96875,
+ "pos_y": -13.28125,
+ "pos_z": 74.84375,
+ "stations": "Wells Dock"
+ },
+ {
+ "name": "Juipedun",
+ "pos_x": -90.34375,
+ "pos_y": 39.75,
+ "pos_z": -61.375,
+ "stations": "Cantor Gateway,Kozin Hub,Phillips' Inheritance,Pratchett Base,Chebyshev Vision,Lee Prospect,Marley Landing,Thompson Base,Barreiros Point"
+ },
+ {
+ "name": "Juipeduwona",
+ "pos_x": 76.46875,
+ "pos_y": 49.46875,
+ "pos_z": 140.34375,
+ "stations": "Collins Terminal,Koontz Settlement"
+ },
+ {
+ "name": "Juipek",
+ "pos_x": 55.4375,
+ "pos_y": -6.21875,
+ "pos_z": 84.5,
+ "stations": "Walter Ring,Bacon Gateway,Zhen Landing,Houssay Hub"
+ },
+ {
+ "name": "Juiquan",
+ "pos_x": 7.3125,
+ "pos_y": -13.6875,
+ "pos_z": -41.90625,
+ "stations": "Nelson Relay,Hurston Hangar,Glashow Terminal"
+ },
+ {
+ "name": "Jukagha",
+ "pos_x": 100,
+ "pos_y": 58.96875,
+ "pos_z": 99.21875,
+ "stations": "Fraas Orbital,Barry Ring"
+ },
+ {
+ "name": "Jukarisagi",
+ "pos_x": -36.5625,
+ "pos_y": -119.25,
+ "pos_z": 20.0625,
+ "stations": "Lysenko Relay,Heinlein Settlement,Wankel Survey"
+ },
+ {
+ "name": "Jukat",
+ "pos_x": -90.65625,
+ "pos_y": 89.96875,
+ "pos_z": -16.34375,
+ "stations": "Mouhot Colony,Herndon Orbital"
+ },
+ {
+ "name": "Jukate",
+ "pos_x": -35.84375,
+ "pos_y": 103.03125,
+ "pos_z": 25.8125,
+ "stations": "Fast Works,Cadamosto Orbital,McKay Hub,Rondon Works"
+ },
+ {
+ "name": "Jukatiwa",
+ "pos_x": -62.84375,
+ "pos_y": -124.90625,
+ "pos_z": 95.875,
+ "stations": "Meikle Terminal,Petaja Vista"
+ },
+ {
+ "name": "Juku",
+ "pos_x": -141.65625,
+ "pos_y": -42.46875,
+ "pos_z": 35.96875,
+ "stations": "Ings Colony,Harrison's Pride"
+ },
+ {
+ "name": "Jukub Huan",
+ "pos_x": -84.46875,
+ "pos_y": -6.1875,
+ "pos_z": 157.4375,
+ "stations": "Barr Terminal,Williams Mines,Jenkinson Works"
+ },
+ {
+ "name": "Jukuju",
+ "pos_x": -93.75,
+ "pos_y": 81.78125,
+ "pos_z": -97.78125,
+ "stations": "Weiss Hub"
+ },
+ {
+ "name": "Jukuna",
+ "pos_x": -37.21875,
+ "pos_y": -26.90625,
+ "pos_z": 116.90625,
+ "stations": "Hammond Camp"
+ },
+ {
+ "name": "Jukundji",
+ "pos_x": 127.3125,
+ "pos_y": -128.1875,
+ "pos_z": 72.78125,
+ "stations": "Takamizawa Prospect,Fujikawa Plant,Vyssotsky Platform,van der Riet Woolley Vision,Dirichlet's Progress"
+ },
+ {
+ "name": "Jukurang",
+ "pos_x": 26.84375,
+ "pos_y": 174.28125,
+ "pos_z": 17.1875,
+ "stations": "Judson Ring,Minkowski Hub"
+ },
+ {
+ "name": "Jula",
+ "pos_x": 173.125,
+ "pos_y": -63.125,
+ "pos_z": 69.09375,
+ "stations": "Marsden Terminal,Laurent Vision,Alfven Port,Cremona Survey"
+ },
+ {
+ "name": "Julahau",
+ "pos_x": 73.15625,
+ "pos_y": -130.875,
+ "pos_z": -90.625,
+ "stations": "Gustav Sporer Platform,Palisa Settlement,Cowell Survey"
+ },
+ {
+ "name": "Julana",
+ "pos_x": -55.84375,
+ "pos_y": -34.03125,
+ "pos_z": 107.90625,
+ "stations": "Carver Silo,Shaikh Port"
+ },
+ {
+ "name": "Julanggarri",
+ "pos_x": 19.21875,
+ "pos_y": 6.40625,
+ "pos_z": -64.65625,
+ "stations": "Willis City,Romanenko Gateway,Metcalf Depot"
+ },
+ {
+ "name": "Julanito",
+ "pos_x": 113.4375,
+ "pos_y": 54.9375,
+ "pos_z": 58.75,
+ "stations": "Dias Reformatory,Gibson Penitentiary"
+ },
+ {
+ "name": "Julannsa",
+ "pos_x": -22.59375,
+ "pos_y": -38.1875,
+ "pos_z": -67.6875,
+ "stations": "Cayley Dock"
+ },
+ {
+ "name": "Julastii",
+ "pos_x": 148.90625,
+ "pos_y": -190.46875,
+ "pos_z": 53,
+ "stations": "Constantine Penal colony,Esposito Vision,Behnisch Terminal"
+ },
+ {
+ "name": "Julasto",
+ "pos_x": -102.59375,
+ "pos_y": -25.96875,
+ "pos_z": -137.21875,
+ "stations": "Bamford Mine"
+ },
+ {
+ "name": "Julu",
+ "pos_x": -60.34375,
+ "pos_y": -184.0625,
+ "pos_z": -0.875,
+ "stations": "Elmore Station,Cowell Orbital,Suntzeff Gateway,Ilyushin Hub"
+ },
+ {
+ "name": "Julung",
+ "pos_x": 4.65625,
+ "pos_y": -122.375,
+ "pos_z": 9.5625,
+ "stations": "Herschel Installation,Millosevich Colony,Noon Barracks"
+ },
+ {
+ "name": "Juma",
+ "pos_x": -34.3125,
+ "pos_y": -51.9375,
+ "pos_z": 65.5625,
+ "stations": "Illy Dock,Moseley Settlement,Herbert Oasis"
+ },
+ {
+ "name": "Jumadis",
+ "pos_x": 62,
+ "pos_y": -20.6875,
+ "pos_z": 44.625,
+ "stations": "Drzewiecki Landing,Varley Hangar,Jones Survey"
+ },
+ {
+ "name": "Jumana",
+ "pos_x": -71.46875,
+ "pos_y": -74.5625,
+ "pos_z": -12.96875,
+ "stations": "Larson Station,Ordway Hangar,Janes' Progress,Soukup Hangar"
+ },
+ {
+ "name": "Jumukulayon",
+ "pos_x": -81.59375,
+ "pos_y": -74.90625,
+ "pos_z": 69.75,
+ "stations": "Laird Ring,Valdes Laboratory"
+ },
+ {
+ "name": "Jumukun",
+ "pos_x": -33.5,
+ "pos_y": -94.59375,
+ "pos_z": -75.4375,
+ "stations": "Faraday Plant,Schwann Holdings"
+ },
+ {
+ "name": "Jumuzgo",
+ "pos_x": 93.96875,
+ "pos_y": -125.21875,
+ "pos_z": 31.15625,
+ "stations": "Andoyer Station,Pond Ring,Artsutanov Station,Ulloa City,Lindstrand Gateway,Nikitin Depot,Maughmer Holdings,Woodroffe Dock,Vess Hub,Lintott Port,McQuarrie Vision"
+ },
+ {
+ "name": "Jun Wenates",
+ "pos_x": 103.53125,
+ "pos_y": -120.6875,
+ "pos_z": -76.09375,
+ "stations": "van Royen Platform,Lehtonen Depot"
+ },
+ {
+ "name": "Junda",
+ "pos_x": 45.96875,
+ "pos_y": -25.46875,
+ "pos_z": 65.5,
+ "stations": "Humphreys Port"
+ },
+ {
+ "name": "Junga",
+ "pos_x": -17.09375,
+ "pos_y": -84.375,
+ "pos_z": 98.15625,
+ "stations": "Samos Orbital,Curtiss Station,Bessel Terminal,Bradshaw Observatory,Lyne Port,Serling's Claim,Henderson Station,Naburimannu Dock,Frobisher Prospect,Guin Town,Vries Silo,Friedman Port"
+ },
+ {
+ "name": "Jungkurara",
+ "pos_x": -161.6875,
+ "pos_y": 22.65625,
+ "pos_z": -42.0625,
+ "stations": "Brooks' Folly,Rodrigues Settlement,Smith Depot"
+ },
+ {
+ "name": "Juniper",
+ "pos_x": -9557.9375,
+ "pos_y": -911.59375,
+ "pos_z": 19806.0625,
+ "stations": "Marigold City"
+ },
+ {
+ "name": "Jupaga",
+ "pos_x": 156.21875,
+ "pos_y": -38.125,
+ "pos_z": 21.21875,
+ "stations": "Milnor Keep,Ghez Stop"
+ },
+ {
+ "name": "Jupagalk",
+ "pos_x": 64.5625,
+ "pos_y": -130.78125,
+ "pos_z": -54.03125,
+ "stations": "Oshima Port"
+ },
+ {
+ "name": "Jupali",
+ "pos_x": -60.03125,
+ "pos_y": 60.71875,
+ "pos_z": 36.8125,
+ "stations": "Blaha Ring,Williams Prospect"
+ },
+ {
+ "name": "Jupalo",
+ "pos_x": -8.03125,
+ "pos_y": -168.0625,
+ "pos_z": 110.53125,
+ "stations": "Gorey Landing,Fabricius Mines,Dyr Barracks"
+ },
+ {
+ "name": "Jupaloku",
+ "pos_x": 45.71875,
+ "pos_y": 107.9375,
+ "pos_z": -52,
+ "stations": "Ford Ring,Weston Port,Bhabha Holdings,Burnell Enterprise,Pirsan Port,Lister Port,Dobzhansky Station,Burbank Enterprise,Vetulani Orbital,Stanier Terminal,Meyrink Gateway,Matthews' Inheritance,Rotsler Holdings,Thoreau Base"
+ },
+ {
+ "name": "Jupang",
+ "pos_x": 211.78125,
+ "pos_y": -116.03125,
+ "pos_z": 28.5,
+ "stations": "Hafner Station,Salam Prospect,Pordenone Prospect"
+ },
+ {
+ "name": "Jupangati",
+ "pos_x": 187.46875,
+ "pos_y": -120.5,
+ "pos_z": 2.09375,
+ "stations": "Kimura Port"
+ },
+ {
+ "name": "Jupanisage",
+ "pos_x": 43.09375,
+ "pos_y": -204.59375,
+ "pos_z": 18.875,
+ "stations": "McDermott Hub,Angstrom Gateway,van Houten Beacon,Bradley Point,Mieville Terminal"
+ },
+ {
+ "name": "Juparoin",
+ "pos_x": 75.78125,
+ "pos_y": 68.21875,
+ "pos_z": 62.65625,
+ "stations": "Fraas Depot,Durrance Landing,Cremona Works"
+ },
+ {
+ "name": "Jura",
+ "pos_x": 139.25,
+ "pos_y": -83.125,
+ "pos_z": -57.46875,
+ "stations": "Eggleton Platform,Baynes Terminal,Brorsen Installation,Bates Bastion,de Caminha Beacon"
+ },
+ {
+ "name": "Jura Laima",
+ "pos_x": -162.375,
+ "pos_y": -30.375,
+ "pos_z": -9.375,
+ "stations": "Borlaug Ring"
+ },
+ {
+ "name": "Jura Lungs",
+ "pos_x": 17,
+ "pos_y": -159.0625,
+ "pos_z": 118.03125,
+ "stations": "Valigursky Settlement,Biesbroeck Orbital,Safdie Enterprise,De Platform"
+ },
+ {
+ "name": "Juracame",
+ "pos_x": 4.34375,
+ "pos_y": 133.625,
+ "pos_z": 41.40625,
+ "stations": "Shunn Platform"
+ },
+ {
+ "name": "Juradhis",
+ "pos_x": 61.53125,
+ "pos_y": -221.25,
+ "pos_z": 120.78125,
+ "stations": "Mead's Claim"
+ },
+ {
+ "name": "Juragura",
+ "pos_x": 134.3125,
+ "pos_y": 75.65625,
+ "pos_z": 77.46875,
+ "stations": "Cook Refinery,Dedman Landing,Rangarajan Refinery"
+ },
+ {
+ "name": "Jurasmat",
+ "pos_x": 30.75,
+ "pos_y": -184.375,
+ "pos_z": 120.84375,
+ "stations": "Lindblad Station,Gaughan Orbital,Brooks Plant,Heinkel Port,Plancius Observatory"
+ },
+ {
+ "name": "Jurati",
+ "pos_x": 38.21875,
+ "pos_y": 4.71875,
+ "pos_z": 115.46875,
+ "stations": "Irwin Vision,Lawson Dock"
+ },
+ {
+ "name": "Jurawadbad",
+ "pos_x": -52.125,
+ "pos_y": 51.875,
+ "pos_z": 71.375,
+ "stations": "Dyson's Claim,Duckworth Dock,Grassmann Vision"
+ },
+ {
+ "name": "Jurcians",
+ "pos_x": 171.5625,
+ "pos_y": -75.125,
+ "pos_z": 59.03125,
+ "stations": "Chernykh Stop"
+ },
+ {
+ "name": "Juro",
+ "pos_x": 63.28125,
+ "pos_y": 13.65625,
+ "pos_z": -181.8125,
+ "stations": "Russell Mine,Pellegrino Penal colony"
+ },
+ {
+ "name": "Jurokkju",
+ "pos_x": 87.25,
+ "pos_y": -69.09375,
+ "pos_z": 27.5625,
+ "stations": "Ohain Survey,Nakamura Port,Chretien Oudemans Survey,Dassault Penal colony,Lynn Legacy"
+ },
+ {
+ "name": "Jurovii",
+ "pos_x": -169.71875,
+ "pos_y": -0.0625,
+ "pos_z": 61.625,
+ "stations": "Bernoulli Orbital,Freycinet Platform,Hartog Terminal"
+ },
+ {
+ "name": "Juru",
+ "pos_x": -105.1875,
+ "pos_y": 98.71875,
+ "pos_z": -22.8125,
+ "stations": "Bunnell Ring,Gunn Terminal"
+ },
+ {
+ "name": "Jurua",
+ "pos_x": -38.6875,
+ "pos_y": -14.09375,
+ "pos_z": -71.0625,
+ "stations": "Beregovoi City,Feustel Port"
+ },
+ {
+ "name": "Juruing",
+ "pos_x": -101.375,
+ "pos_y": 134.59375,
+ "pos_z": 21.25,
+ "stations": "Beckman Landing"
+ },
+ {
+ "name": "Jurumis",
+ "pos_x": 121.40625,
+ "pos_y": 9.40625,
+ "pos_z": 108.5625,
+ "stations": "Polya Sanctuary"
+ },
+ {
+ "name": "Jurunmi",
+ "pos_x": 8.4375,
+ "pos_y": 85,
+ "pos_z": 78.3125,
+ "stations": "Pike Enterprise,Ziemkiewicz Gateway,Coulomb Station"
+ },
+ {
+ "name": "Jurus",
+ "pos_x": -74.25,
+ "pos_y": 165,
+ "pos_z": -24.28125,
+ "stations": "Samuda Retreat,Plexico Dock"
+ },
+ {
+ "name": "Jurusasar",
+ "pos_x": 13.8125,
+ "pos_y": -205.28125,
+ "pos_z": 27.65625,
+ "stations": "Kosai Horizons,Lyne Reach"
+ },
+ {
+ "name": "K Camelopardalis",
+ "pos_x": -45.21875,
+ "pos_y": 26.625,
+ "pos_z": -43.46875,
+ "stations": "Payette Hub,Foda Orbital,Pasteur Prospect"
+ },
+ {
+ "name": "k Velorum A",
+ "pos_x": 161.75,
+ "pos_y": 23.3125,
+ "pos_z": -20,
+ "stations": "Godwin Port,Anthony Point"
+ },
+ {
+ "name": "K'uanele",
+ "pos_x": 69.78125,
+ "pos_y": 71.15625,
+ "pos_z": -68.34375,
+ "stations": "Cartan Station,Van Scyoc Hub"
+ },
+ {
+ "name": "K'uei Zu",
+ "pos_x": -13,
+ "pos_y": -191.40625,
+ "pos_z": -6.8125,
+ "stations": "Tange Platform,Winthrop Hub,Oterma Holdings"
+ },
+ {
+ "name": "K'unchadrus",
+ "pos_x": 59.46875,
+ "pos_y": 16.09375,
+ "pos_z": 67.0625,
+ "stations": "Selye Installation,Godwin Plant"
+ },
+ {
+ "name": "Ka'apok",
+ "pos_x": 63.40625,
+ "pos_y": -196.5625,
+ "pos_z": -61.28125,
+ "stations": "Chelomey Port"
+ },
+ {
+ "name": "Kaal",
+ "pos_x": -18.34375,
+ "pos_y": 66.71875,
+ "pos_z": -21.59375,
+ "stations": "Vercors Station,Anderson Camp"
+ },
+ {
+ "name": "Kaan",
+ "pos_x": 53.9375,
+ "pos_y": -139.1875,
+ "pos_z": 148.28125,
+ "stations": "Lippisch Terminal,Hammel Port,Anderson Hub,Dalgarno's Inheritance"
+ },
+ {
+ "name": "Kaana",
+ "pos_x": -91.28125,
+ "pos_y": 70.625,
+ "pos_z": -48.53125,
+ "stations": "Fuca Platform"
+ },
+ {
+ "name": "Kaang Wa",
+ "pos_x": 66.625,
+ "pos_y": -155,
+ "pos_z": 73.59375,
+ "stations": "Thomas Dock,Hannu Arena"
+ },
+ {
+ "name": "Kab",
+ "pos_x": -24.59375,
+ "pos_y": -104.53125,
+ "pos_z": -32.4375,
+ "stations": "Schweickart Orbital,Aristotle's Inheritance,Fujimori Orbital"
+ },
+ {
+ "name": "Kaba",
+ "pos_x": 3.09375,
+ "pos_y": 103.25,
+ "pos_z": 23.46875,
+ "stations": "Robinson Station,Moriarty Mine,Nelder's Progress"
+ },
+ {
+ "name": "Kabiak",
+ "pos_x": 150.71875,
+ "pos_y": -180.875,
+ "pos_z": -10.53125,
+ "stations": "Filter Base,Hiyya Dock,Shirazi Vision,Knorre Station"
+ },
+ {
+ "name": "Kabijao",
+ "pos_x": -163.125,
+ "pos_y": -2.65625,
+ "pos_z": -44.21875,
+ "stations": "Piercy Horizons,Yu Orbital,Nikitin Port,Marlowe Prospect"
+ },
+ {
+ "name": "Kabiku",
+ "pos_x": -34.34375,
+ "pos_y": -24.5,
+ "pos_z": 173.375,
+ "stations": "Bruce Enterprise,Pohl Hub,Efremov Vision"
+ },
+ {
+ "name": "Kabil",
+ "pos_x": -104.1875,
+ "pos_y": 118,
+ "pos_z": 74.46875,
+ "stations": "McArthur Terminal,Ramon Settlement,Hedley Hub,Dorsey Hub,Savery Depot"
+ },
+ {
+ "name": "Kabuga",
+ "pos_x": 79.71875,
+ "pos_y": -21.59375,
+ "pos_z": 35.125,
+ "stations": "Schroeder Installation,Shepard Platform,Pournelle Hub"
+ },
+ {
+ "name": "Kacha Wit",
+ "pos_x": 136.375,
+ "pos_y": 29.46875,
+ "pos_z": 35.8125,
+ "stations": "Noon's Progress,Fozard Installation"
+ },
+ {
+ "name": "Kachakapa",
+ "pos_x": 33.96875,
+ "pos_y": -51.75,
+ "pos_z": -66.5,
+ "stations": "Heceta's Inheritance,Khrenov Dock,Bisnovatyi-Kogan Installation"
+ },
+ {
+ "name": "Kachatrimih",
+ "pos_x": -58.625,
+ "pos_y": 127.8125,
+ "pos_z": 25.03125,
+ "stations": "Stillman Station,Ostrander Installation"
+ },
+ {
+ "name": "Kachi Buj",
+ "pos_x": 0.875,
+ "pos_y": -238.78125,
+ "pos_z": 20.5,
+ "stations": "Zaschka Outpost"
+ },
+ {
+ "name": "Kachian",
+ "pos_x": 25.25,
+ "pos_y": -44.65625,
+ "pos_z": -25.96875,
+ "stations": "Goddard Station,Bernard City,Eratosthenes Hub,Avogadro Plant,Lundwall Landing"
+ },
+ {
+ "name": "Kachinas",
+ "pos_x": -88.875,
+ "pos_y": -76.09375,
+ "pos_z": -34.34375,
+ "stations": "Coats Plant,Evans Dock,Krusvar Arsenal"
+ },
+ {
+ "name": "Kachiri",
+ "pos_x": 59.5625,
+ "pos_y": -17.625,
+ "pos_z": -71.34375,
+ "stations": "Burckhardt Gateway,Van Scyoc Vision,Waldrop Hub,Grassmann Installation,Doi Enterprise"
+ },
+ {
+ "name": "Kachirigin",
+ "pos_x": -105.375,
+ "pos_y": -73.34375,
+ "pos_z": 27.75,
+ "stations": "Nowak Orbital,Schrodinger Terminal,Zebrowski Installation,Rukavishnikov City,Dana Base,Sleator Silo,Berezin Installation"
+ },
+ {
+ "name": "Kachuahari",
+ "pos_x": -74.03125,
+ "pos_y": -127.53125,
+ "pos_z": -24.34375,
+ "stations": "Stiegler Station"
+ },
+ {
+ "name": "Kacobog",
+ "pos_x": 98.90625,
+ "pos_y": -21.9375,
+ "pos_z": 64.875,
+ "stations": "Bennett Landing,Snyder Refinery"
+ },
+ {
+ "name": "Kacomam",
+ "pos_x": 84.4375,
+ "pos_y": -48.5,
+ "pos_z": -27.84375,
+ "stations": "Goldberg City,Kennan Beacon"
+ },
+ {
+ "name": "Kada",
+ "pos_x": -73.28125,
+ "pos_y": 62.25,
+ "pos_z": 89.28125,
+ "stations": "Baudry Terminal,Thiele Plant,Rennie Mines"
+ },
+ {
+ "name": "Kadaren",
+ "pos_x": -112.25,
+ "pos_y": -75.65625,
+ "pos_z": 54.0625,
+ "stations": "Hyecho Holdings,Gaspar de Lemos Relay,Vasquez de Coronado Vista"
+ },
+ {
+ "name": "Kadi",
+ "pos_x": -13.78125,
+ "pos_y": -32.8125,
+ "pos_z": -40.9375,
+ "stations": "Marshburn Station,Fisher Prospect,Bennett Enterprise"
+ },
+ {
+ "name": "Kadicat",
+ "pos_x": -37.0625,
+ "pos_y": -102.4375,
+ "pos_z": 76.3125,
+ "stations": "Potagos Installation,Harrison Stop"
+ },
+ {
+ "name": "Kadj Wen",
+ "pos_x": -35.46875,
+ "pos_y": -60.75,
+ "pos_z": 148.65625,
+ "stations": "Santos Plant,Barentsz Prospect"
+ },
+ {
+ "name": "Kadjerong",
+ "pos_x": -52.5,
+ "pos_y": -69.5,
+ "pos_z": -30.375,
+ "stations": "Xiaoguan Orbital,Qurra Horizons"
+ },
+ {
+ "name": "Kadroa",
+ "pos_x": -55.4375,
+ "pos_y": -0.59375,
+ "pos_z": 177.1875,
+ "stations": "Macan Station,Banks Vision,Rawn Settlement"
+ },
+ {
+ "name": "Kadrusa",
+ "pos_x": 37.8125,
+ "pos_y": -120.28125,
+ "pos_z": -1.34375,
+ "stations": "Slipher Vision,O'Leary Terminal,Kahn Holdings"
+ },
+ {
+ "name": "Kadu mist",
+ "pos_x": -48.3125,
+ "pos_y": -4.875,
+ "pos_z": 85.5625,
+ "stations": "Burroughs City,Stephens City,Shosuke Gateway,Yolen Gateway,Ahmed Enterprise,Darnielle Terminal,Harrison Legacy"
+ },
+ {
+ "name": "Kadubres",
+ "pos_x": 57.90625,
+ "pos_y": 31,
+ "pos_z": -97,
+ "stations": "Otto's Claim"
+ },
+ {
+ "name": "Kadunet",
+ "pos_x": 38.5,
+ "pos_y": 1.9375,
+ "pos_z": -122.46875,
+ "stations": "Ford Hub,Pinto Survey,Farkas Palace"
+ },
+ {
+ "name": "Kaduvumbaa",
+ "pos_x": 84.6875,
+ "pos_y": 58.8125,
+ "pos_z": -17.25,
+ "stations": "North Enterprise,Bulgarin Ring,Wagner Beacon,Morrison Orbital,Bierce Barracks"
+ },
+ {
+ "name": "Kafigelabon",
+ "pos_x": 18.75,
+ "pos_y": -77.3125,
+ "pos_z": 67.03125,
+ "stations": "Hillary Port,Schachner Refinery"
+ },
+ {
+ "name": "Kafigenon",
+ "pos_x": 139.28125,
+ "pos_y": 49.78125,
+ "pos_z": 22,
+ "stations": "Crown Depot,Derleth Terminal,Petaja City,Crowley Hub"
+ },
+ {
+ "name": "Kagudi",
+ "pos_x": -47.6875,
+ "pos_y": -42.96875,
+ "pos_z": -77.65625,
+ "stations": "Williamson Dock,Scalzi Silo,Hilmers Base"
+ },
+ {
+ "name": "Kagutine",
+ "pos_x": 105.4375,
+ "pos_y": 24.96875,
+ "pos_z": 66.15625,
+ "stations": "Morukov Stop,Spinrad Survey"
+ },
+ {
+ "name": "Kagutsuchi",
+ "pos_x": -11.5,
+ "pos_y": -84.5625,
+ "pos_z": -162.65625,
+ "stations": "Crown Prospect,Walker Survey,Dantec Terminal,Crichton Palace,Barjavel Hub"
+ },
+ {
+ "name": "Kaha'i",
+ "pos_x": -7.03125,
+ "pos_y": 60.8125,
+ "pos_z": -21.8125,
+ "stations": "Humphreys Beacon,Leiber City,Wells Settlement,Brust Installation"
+ },
+ {
+ "name": "Kaia Bajaja",
+ "pos_x": -16.71875,
+ "pos_y": -6.1875,
+ "pos_z": 120.84375,
+ "stations": "Brin Enterprise,Howe Landing,Krusvar Platform"
+ },
+ {
+ "name": "Kaiabi",
+ "pos_x": -120.28125,
+ "pos_y": 96.03125,
+ "pos_z": 71.40625,
+ "stations": "Gaspar de Portola Colony,Drzewiecki Mines"
+ },
+ {
+ "name": "Kaiablingah",
+ "pos_x": -105,
+ "pos_y": -106.84375,
+ "pos_z": 71.8125,
+ "stations": "Cady Landing"
+ },
+ {
+ "name": "Kaiakul",
+ "pos_x": 78.59375,
+ "pos_y": -129.59375,
+ "pos_z": 33.4375,
+ "stations": "Barlowe Station,Ashbrook Terminal,Bliss Gateway,Takamizawa Hub"
+ },
+ {
+ "name": "Kaiawal",
+ "pos_x": 144.25,
+ "pos_y": -12.8125,
+ "pos_z": 69.84375,
+ "stations": "Kelly Point,Toll Port"
+ },
+ {
+ "name": "Kainango",
+ "pos_x": 16.4375,
+ "pos_y": -159.71875,
+ "pos_z": 111.6875,
+ "stations": "William Sargent Outpost"
+ },
+ {
+ "name": "Kairi",
+ "pos_x": 137.59375,
+ "pos_y": -17.15625,
+ "pos_z": -37.375,
+ "stations": "Carleson Hub,Galindo Port,Bridges Terminal,Wiener Dock,Springer Enterprise,Saavedra Hub,Davidson Enterprise,Williamson's Folly,Carver Orbital,Piserchia's Claim"
+ },
+ {
+ "name": "Kairos",
+ "pos_x": 26.875,
+ "pos_y": -4.09375,
+ "pos_z": 51.5,
+ "stations": "Boodt Dock,Vaucanson Platform,Fossum Colony,Bernoulli Penal colony,Matheson Relay"
+ },
+ {
+ "name": "Kairrodo",
+ "pos_x": 23.875,
+ "pos_y": 77.78125,
+ "pos_z": -130.3125,
+ "stations": "Yu Dock,Scott Beacon"
+ },
+ {
+ "name": "Kaites",
+ "pos_x": -40.40625,
+ "pos_y": -50.65625,
+ "pos_z": -152.09375,
+ "stations": "Converse Prospect"
+ },
+ {
+ "name": "Kaititja",
+ "pos_x": -143.59375,
+ "pos_y": -5.65625,
+ "pos_z": -57.46875,
+ "stations": "Abnett's Inheritance,Riemann Reformatory,Crowley Enterprise"
+ },
+ {
+ "name": "Kaitsan",
+ "pos_x": 14.40625,
+ "pos_y": 73.875,
+ "pos_z": -109.5625,
+ "stations": "McCulley Relay,Guidoni Legacy"
+ },
+ {
+ "name": "Kaiwarabak",
+ "pos_x": 63.90625,
+ "pos_y": 10.78125,
+ "pos_z": 77.8125,
+ "stations": "Nowak Platform,Mayer Horizons"
+ },
+ {
+ "name": "Kajuku",
+ "pos_x": -9525.03125,
+ "pos_y": -943.40625,
+ "pos_z": 19795.5625,
+ "stations": "Prince Kajukus Paradise"
+ },
+ {
+ "name": "Kakan",
+ "pos_x": 80.5625,
+ "pos_y": 23.9375,
+ "pos_z": 143.6875,
+ "stations": "Clebsch Vision,Pinzon Beacon"
+ },
+ {
+ "name": "Kakas",
+ "pos_x": -65.78125,
+ "pos_y": -22.4375,
+ "pos_z": 78.46875,
+ "stations": "Kube-McDowell Enterprise,Reynolds Gateway,Robinson Beacon,Zajdel Base"
+ },
+ {
+ "name": "Kakatha",
+ "pos_x": -18.71875,
+ "pos_y": -121.46875,
+ "pos_z": -53,
+ "stations": "Bahcall City,Grigorovich Installation,Roberts Landing"
+ },
+ {
+ "name": "Kakau",
+ "pos_x": 18.46875,
+ "pos_y": -110.8125,
+ "pos_z": 116.84375,
+ "stations": "Bohrmann Relay"
+ },
+ {
+ "name": "Kakmbul",
+ "pos_x": 54.40625,
+ "pos_y": 13.84375,
+ "pos_z": -56.8125,
+ "stations": "Haise Relay,Bacon Hub,Conway Base,Sinclair Station,Balandin Enterprise"
+ },
+ {
+ "name": "Kakmburra",
+ "pos_x": 102.78125,
+ "pos_y": -115.90625,
+ "pos_z": 101.40625,
+ "stations": "Chaffee Vision,Hale Dock,Bauschinger Terminal,Mandel Gateway,Louis de Lacaille Arsenal,Anastase Perrotin Station,Morgan Enterprise,Pytheas Exchange,Arrhenius Hub,Moore Dock,Theodor Winnecke Base,Clebsch's Pride,Truly Vision,Hoffmeister Dock,Borrego Port"
+ },
+ {
+ "name": "Kakmburu",
+ "pos_x": -80.375,
+ "pos_y": -48.5625,
+ "pos_z": -12.21875,
+ "stations": "Kneale Gateway,Obruchev Horizons,Garnier Dock,Lambert Plant,Gerrold Enterprise"
+ },
+ {
+ "name": "Kakmbutan",
+ "pos_x": -112.1875,
+ "pos_y": -106.46875,
+ "pos_z": 36.28125,
+ "stations": "Macgregor Orbital,Preuss Enterprise,Gessi Station,Fiennes Point"
+ },
+ {
+ "name": "Kala",
+ "pos_x": -2.90625,
+ "pos_y": 5.59375,
+ "pos_z": 61.1875,
+ "stations": "Fischer Station,Carey Vision,Pauling City,Kovalevsky Installation"
+ },
+ {
+ "name": "Kalaa",
+ "pos_x": 147.375,
+ "pos_y": 31.3125,
+ "pos_z": 34.75,
+ "stations": "Gallun Prospect"
+ },
+ {
+ "name": "Kalaako",
+ "pos_x": -82.15625,
+ "pos_y": 18.90625,
+ "pos_z": -70.0625,
+ "stations": "Pennington City"
+ },
+ {
+ "name": "Kalads",
+ "pos_x": 82.3125,
+ "pos_y": -22.53125,
+ "pos_z": -151.40625,
+ "stations": "Schweickart Settlement,Bloomfield Dock,McKay's Folly"
+ },
+ {
+ "name": "Kalai",
+ "pos_x": 85.5,
+ "pos_y": 42.0625,
+ "pos_z": -21.21875,
+ "stations": "Broderick City,Wright Plant,Macgregor's Folly"
+ },
+ {
+ "name": "Kalaik",
+ "pos_x": 104.34375,
+ "pos_y": -161.5625,
+ "pos_z": 64.78125,
+ "stations": "Ferguson Survey,Pons Survey"
+ },
+ {
+ "name": "Kalak",
+ "pos_x": -54.96875,
+ "pos_y": -73.90625,
+ "pos_z": -68.78125,
+ "stations": "Nobel Terminal,Tavares Vision,Sarrantonio Port,Fisher Vision,Abel's Folly"
+ },
+ {
+ "name": "Kalama",
+ "pos_x": 1.46875,
+ "pos_y": -194.09375,
+ "pos_z": -15.28125,
+ "stations": "Barlowe Colony"
+ },
+ {
+ "name": "Kalana",
+ "pos_x": 131.78125,
+ "pos_y": -12.96875,
+ "pos_z": 60.03125,
+ "stations": "Burkin Port,Moore Prospect,Bujold Enterprise,Christopher Mines"
+ },
+ {
+ "name": "Kalangputys",
+ "pos_x": 143.90625,
+ "pos_y": -15.53125,
+ "pos_z": 5.25,
+ "stations": "Carlisle Base"
+ },
+ {
+ "name": "Kalarnuteti",
+ "pos_x": -12.65625,
+ "pos_y": -181.40625,
+ "pos_z": 70,
+ "stations": "Tan Port"
+ },
+ {
+ "name": "Kalasar",
+ "pos_x": 10.875,
+ "pos_y": 79.25,
+ "pos_z": -72,
+ "stations": "Kwolek Hub,Hedley Terminal,Nasmyth Landing,Hopkins Beacon,Thirsk Prospect"
+ },
+ {
+ "name": "Kalatzin",
+ "pos_x": 41.4375,
+ "pos_y": -139.5,
+ "pos_z": 64.59375,
+ "stations": "DiFate Orbital,Danforth Hub,Macan Survey,Wallis Relay"
+ },
+ {
+ "name": "Kalb",
+ "pos_x": -0.5,
+ "pos_y": 44.5,
+ "pos_z": 81.09375,
+ "stations": "Blackwell Orbital,Gidzenko Terminal,Bagian Station,Hobaugh Station,Boswell City,Avdeyev City,Chapman Hub,Maury Point,Burstein Terminal,Mach Port,Lewitt Installation,Hausdorff Relay,Asher Exchange,Reis Holdings,Ehrlich Port"
+ },
+ {
+ "name": "Kaledoni",
+ "pos_x": 55.84375,
+ "pos_y": -61.96875,
+ "pos_z": 79.9375,
+ "stations": "Steele Laboratory,Fung Platform,Currie Enterprise"
+ },
+ {
+ "name": "Kaleke",
+ "pos_x": 48.03125,
+ "pos_y": -16.78125,
+ "pos_z": -157.59375,
+ "stations": "Chalker Hub,Alvarez de Pineda City,Schilling Station,Thompson Enterprise,Tilman Base,Fremont Prospect"
+ },
+ {
+ "name": "Kaleni",
+ "pos_x": 74.1875,
+ "pos_y": -243.0625,
+ "pos_z": 15.375,
+ "stations": "Battani Orbital,Vasquez de Coronado Survey,Anderson City,Nelder Arsenal"
+ },
+ {
+ "name": "Kalenile",
+ "pos_x": 4.0625,
+ "pos_y": 11.40625,
+ "pos_z": -115.9375,
+ "stations": "Watson Beacon,Boswell Retreat"
+ },
+ {
+ "name": "Kaleo",
+ "pos_x": -197.6875,
+ "pos_y": 55.9375,
+ "pos_z": 45.53125,
+ "stations": "Martinez Platform,Moorcock Beacon"
+ },
+ {
+ "name": "Kali",
+ "pos_x": 33.59375,
+ "pos_y": 57.375,
+ "pos_z": -28.84375,
+ "stations": "Kolmogorov City,Hoffman Station,Lerner Terminal,Kolmogorov Beacon,Schmidt Enterprise,Conway Keep"
+ },
+ {
+ "name": "Kali'na",
+ "pos_x": -168.75,
+ "pos_y": -57.375,
+ "pos_z": 32.6875,
+ "stations": "Soto Mines,Krupkat Outpost,Byrd's Inheritance,Burkin Beacon"
+ },
+ {
+ "name": "Kalibia",
+ "pos_x": 128.34375,
+ "pos_y": -14.15625,
+ "pos_z": 59,
+ "stations": "Palmer Terminal"
+ },
+ {
+ "name": "Kaliki",
+ "pos_x": 37.46875,
+ "pos_y": -94.15625,
+ "pos_z": -48.09375,
+ "stations": "Kaluta Prospect,Oren City,Vaucouleurs Hub,Trimble Station,Hodgkinson Survey"
+ },
+ {
+ "name": "Kalikil",
+ "pos_x": 171.71875,
+ "pos_y": -139.0625,
+ "pos_z": -41.78125,
+ "stations": "Morrow Outpost,Messerschmitt Platform,Kennicott Point"
+ },
+ {
+ "name": "Kaline",
+ "pos_x": 47.46875,
+ "pos_y": 28.34375,
+ "pos_z": -96.75,
+ "stations": "Due Station,Fawcett Hub,Baudry's Pride,Taine Gateway,Hutchinson Town"
+ },
+ {
+ "name": "Kalivunian",
+ "pos_x": 167.25,
+ "pos_y": -166.65625,
+ "pos_z": -17.1875,
+ "stations": "Searle Survey,Hansen Port,Struve Colony,West Reach"
+ },
+ {
+ "name": "Kalkaduna",
+ "pos_x": 120.34375,
+ "pos_y": -131.4375,
+ "pos_z": -97.28125,
+ "stations": "Dillon Mines,Jacobi Enterprise,Mattingly Orbital,Krigstein Settlement"
+ },
+ {
+ "name": "Kalkinja",
+ "pos_x": 89.5,
+ "pos_y": 79.1875,
+ "pos_z": -121.84375,
+ "stations": "Bisson Reach"
+ },
+ {
+ "name": "Kalkyri",
+ "pos_x": -127.71875,
+ "pos_y": -18.375,
+ "pos_z": 42.6875,
+ "stations": "Voss Colony"
+ },
+ {
+ "name": "Kalufon",
+ "pos_x": -114.375,
+ "pos_y": -104.0625,
+ "pos_z": 39.59375,
+ "stations": "Slayton Terminal,Korniyenko Base"
+ },
+ {
+ "name": "Kaluhet",
+ "pos_x": 64.03125,
+ "pos_y": 87.625,
+ "pos_z": 41.28125,
+ "stations": "Yaping Hub,Herschel Orbital"
+ },
+ {
+ "name": "Kalumar",
+ "pos_x": -29.34375,
+ "pos_y": -1.40625,
+ "pos_z": 90.6875,
+ "stations": "Andreas Ring,Runco Port"
+ },
+ {
+ "name": "Kalunkul",
+ "pos_x": -0.1875,
+ "pos_y": -135.9375,
+ "pos_z": 127.28125,
+ "stations": "Ryle's Claim,Farmer Observatory"
+ },
+ {
+ "name": "Kalva",
+ "pos_x": 101.90625,
+ "pos_y": 95.4375,
+ "pos_z": 38.4375,
+ "stations": "Moore Plant,McCaffrey Platform"
+ },
+ {
+ "name": "Kalvaitu",
+ "pos_x": -7.21875,
+ "pos_y": -150.59375,
+ "pos_z": -10.8125,
+ "stations": "Fox Holdings,Suydam Point,Werber's Inheritance"
+ },
+ {
+ "name": "Kalvante",
+ "pos_x": 118.78125,
+ "pos_y": -162.8125,
+ "pos_z": -13.96875,
+ "stations": "Vlaicu Station"
+ },
+ {
+ "name": "Kalveig",
+ "pos_x": 64.4375,
+ "pos_y": 62.90625,
+ "pos_z": 74.0625,
+ "stations": "Schweickart Works,Rushd Holdings"
+ },
+ {
+ "name": "Kalvelis",
+ "pos_x": 130.375,
+ "pos_y": -10.46875,
+ "pos_z": -9.09375,
+ "stations": "Beekman Gateway,Pordenone Mines,Stairs Station,Brooks Laboratory,Sternberg Beacon"
+ },
+ {
+ "name": "Kalveti",
+ "pos_x": -7.96875,
+ "pos_y": -15.65625,
+ "pos_z": -121.40625,
+ "stations": "Krikalev Ring,Lichtenberg Ring"
+ },
+ {
+ "name": "Kamadhenu",
+ "pos_x": 110,
+ "pos_y": -99.96875,
+ "pos_z": -13.375,
+ "stations": "Shajn Market,Couper Hub"
+ },
+ {
+ "name": "Kamahu",
+ "pos_x": -89.5625,
+ "pos_y": 12.15625,
+ "pos_z": -148.96875,
+ "stations": "Elion Platform,Sullivan Oasis"
+ },
+ {
+ "name": "Kamaki",
+ "pos_x": 87.5625,
+ "pos_y": -20.90625,
+ "pos_z": 85.96875,
+ "stations": "Shinjo Station,Kojima Gateway"
+ },
+ {
+ "name": "Kamandu",
+ "pos_x": -125.125,
+ "pos_y": 78.375,
+ "pos_z": -113.96875,
+ "stations": "Converse Port,Holberg Enterprise,Young Gateway,Thompson Survey,Carrasco Point"
+ },
+ {
+ "name": "Kamarada",
+ "pos_x": 97.125,
+ "pos_y": -3.84375,
+ "pos_z": 0.84375,
+ "stations": "Papin Outpost,Baudry Prospect,Kregel Refinery"
+ },
+ {
+ "name": "Kamas",
+ "pos_x": 137.625,
+ "pos_y": -37.8125,
+ "pos_z": 49.96875,
+ "stations": "Beg Terminal,Grigson Base,Davis Camp,Goodricke Vision"
+ },
+ {
+ "name": "Kamaskapus",
+ "pos_x": 60.21875,
+ "pos_y": -164.625,
+ "pos_z": -51.28125,
+ "stations": "Shea Landing,Gonnessiat Base,Gordon Base"
+ },
+ {
+ "name": "Kamato",
+ "pos_x": -138.90625,
+ "pos_y": -84.84375,
+ "pos_z": -16.46875,
+ "stations": "Campbell Sanctuary,Stillman Escape"
+ },
+ {
+ "name": "Kamba",
+ "pos_x": 156.5,
+ "pos_y": -70.0625,
+ "pos_z": 49.0625,
+ "stations": "Tuttle Survey,Dixon Colony"
+ },
+ {
+ "name": "Kambaiambo",
+ "pos_x": 44.53125,
+ "pos_y": -17.8125,
+ "pos_z": 138.21875,
+ "stations": "Vonarburg Platform,Fernao do Po Prospect"
+ },
+ {
+ "name": "Kambala",
+ "pos_x": 43.78125,
+ "pos_y": 19.4375,
+ "pos_z": -99.9375,
+ "stations": "Babbage Orbital,Payne Arsenal,Fisk's Progress"
+ },
+ {
+ "name": "Kambarci",
+ "pos_x": 116.0625,
+ "pos_y": 60.46875,
+ "pos_z": 104,
+ "stations": "Piercy City,Young Port,Stjepan Seljan Silo"
+ },
+ {
+ "name": "Kambila",
+ "pos_x": -96.03125,
+ "pos_y": -47.71875,
+ "pos_z": -49.59375,
+ "stations": "Gurney Oasis,Shepard Base,Bosch Reserve"
+ },
+ {
+ "name": "Kambo",
+ "pos_x": 30.0625,
+ "pos_y": -130.96875,
+ "pos_z": -65.84375,
+ "stations": "Wenzel Terminal,Hoffleit Hub"
+ },
+ {
+ "name": "Kambul",
+ "pos_x": 131.65625,
+ "pos_y": -195.96875,
+ "pos_z": 58,
+ "stations": "Lyot Colony,Yuzhe Settlement,Oramus Vision,Orban Prospect"
+ },
+ {
+ "name": "Kamcab",
+ "pos_x": 145.40625,
+ "pos_y": 77.46875,
+ "pos_z": -26.8125,
+ "stations": "MacVicar Camp"
+ },
+ {
+ "name": "Kamcab Yu",
+ "pos_x": 178.78125,
+ "pos_y": -132.40625,
+ "pos_z": -12.90625,
+ "stations": "Kandrup Mine,Maunder Base"
+ },
+ {
+ "name": "Kamcabea",
+ "pos_x": 125.5,
+ "pos_y": 54.71875,
+ "pos_z": 74.4375,
+ "stations": "Hughes Enterprise,Bayley Terminal,Andree Ring,Shalatula Terminal"
+ },
+ {
+ "name": "Kamcabi",
+ "pos_x": 92.28125,
+ "pos_y": 69.3125,
+ "pos_z": 62.46875,
+ "stations": "Kimberlin Settlement,Bujold Plant,Bethke Holdings"
+ },
+ {
+ "name": "Kamcha",
+ "pos_x": 10.3125,
+ "pos_y": -160.53125,
+ "pos_z": 74.1875,
+ "stations": "Saarinen Station,Reeves Hub,Fowler Ring,Kidinnu Gateway,Somayaji Vision,Barlowe Ring,Gurshtein Port,Chertovsky City,Jeschke Beacon,Kosai City,Ortiz Moreno Dock,Rosenberger Depot"
+ },
+ {
+ "name": "Kamchaa",
+ "pos_x": 80.9375,
+ "pos_y": -83.28125,
+ "pos_z": -23.03125,
+ "stations": "Mikulin Hub,Weierstrass Laboratory"
+ },
+ {
+ "name": "Kamchadal",
+ "pos_x": -91.3125,
+ "pos_y": -114.375,
+ "pos_z": -51.09375,
+ "stations": "Walheim Enterprise,Ray Terminal,von Bellingshausen Arsenal,Hiraga Installation"
+ },
+ {
+ "name": "Kamchadals",
+ "pos_x": 170.1875,
+ "pos_y": -119.5,
+ "pos_z": -16.1875,
+ "stations": "Mikoyan Hub,Dalgarno Station,Korlevic Horizons,Holdstock Beacon"
+ },
+ {
+ "name": "Kamchaggama",
+ "pos_x": -46,
+ "pos_y": -214.65625,
+ "pos_z": 53.75,
+ "stations": "Gray Depot"
+ },
+ {
+ "name": "Kamchairra",
+ "pos_x": -51.5,
+ "pos_y": -34.375,
+ "pos_z": -31.59375,
+ "stations": "Lenoir Colony,Napier Orbital"
+ },
+ {
+ "name": "Kamchandika",
+ "pos_x": -104.5,
+ "pos_y": -110.625,
+ "pos_z": 24.03125,
+ "stations": "Fozard Mines,Burnham Hangar,Huberath Station"
+ },
+ {
+ "name": "Kamchansan",
+ "pos_x": 44.8125,
+ "pos_y": -184.6875,
+ "pos_z": 36.3125,
+ "stations": "William Sargent Platform"
+ },
+ {
+ "name": "Kamchata",
+ "pos_x": 35.65625,
+ "pos_y": -15.71875,
+ "pos_z": -70.03125,
+ "stations": "Tuan Platform,Krikalev Gateway,la Cosa Relay,Schweickart Enterprise,Lichtenberg Escape"
+ },
+ {
+ "name": "Kame",
+ "pos_x": 43.28125,
+ "pos_y": 9.875,
+ "pos_z": 65.96875,
+ "stations": "Zewail Mines,Hilmers Port"
+ },
+ {
+ "name": "Kamebis",
+ "pos_x": -161.3125,
+ "pos_y": 15.15625,
+ "pos_z": 74.125,
+ "stations": "Frobisher Mines"
+ },
+ {
+ "name": "Kameni",
+ "pos_x": 55.84375,
+ "pos_y": -28.8125,
+ "pos_z": -59.96875,
+ "stations": "Lucid Orbital"
+ },
+ {
+ "name": "Kamenten",
+ "pos_x": 22.15625,
+ "pos_y": -113.46875,
+ "pos_z": -89.0625,
+ "stations": "Fermat Mine"
+ },
+ {
+ "name": "Kamici",
+ "pos_x": 57.0625,
+ "pos_y": 37.875,
+ "pos_z": 0.75,
+ "stations": "Gaiman Port,Chasles Base,Peary Enterprise,Selberg Survey"
+ },
+ {
+ "name": "Kamil",
+ "pos_x": 68.5625,
+ "pos_y": 35.21875,
+ "pos_z": -179,
+ "stations": "Eskridge's Claim"
+ },
+ {
+ "name": "Kamilagro",
+ "pos_x": -24.65625,
+ "pos_y": 43.375,
+ "pos_z": -129.4375,
+ "stations": "Margulis Orbital,Dzhanibekov Station,Grissom Gateway,Sinisalo Installation"
+ },
+ {
+ "name": "Kamito",
+ "pos_x": 65.3125,
+ "pos_y": -83.4375,
+ "pos_z": -70.15625,
+ "stations": "Newcomb Hub,Digges Station,Kube-McDowell Vision,Potez Port"
+ },
+ {
+ "name": "Kamitra",
+ "pos_x": 5.53125,
+ "pos_y": -183.40625,
+ "pos_z": 63.84375,
+ "stations": "Hammel Terminal,Navigator Reach,Chaviano Enterprise,Cauchy Relay,Westerhout Terminal"
+ },
+ {
+ "name": "Kammaits",
+ "pos_x": -79.8125,
+ "pos_y": 2,
+ "pos_z": 20.875,
+ "stations": "Mukai Hub,Glenn Installation,Messerschmid Keep"
+ },
+ {
+ "name": "Kammamalan",
+ "pos_x": -7.625,
+ "pos_y": -142.875,
+ "pos_z": 100.53125,
+ "stations": "Adams Hub,Morris Arsenal"
+ },
+ {
+ "name": "Kammari",
+ "pos_x": 37.875,
+ "pos_y": 73.9375,
+ "pos_z": 151.59375,
+ "stations": "Crowley Depot,Pribylov Terminal,Wollheim Installation"
+ },
+ {
+ "name": "Kammato",
+ "pos_x": -98.8125,
+ "pos_y": -53.1875,
+ "pos_z": 3.59375,
+ "stations": "Hughes Port,Oliver Dock,O'Brien Enterprise,Grijalva Hub,Zetford Ring,Taine Orbital,North Orbital,Quick Gateway,Webb Palace,Krylov Point"
+ },
+ {
+ "name": "Kamocadroa",
+ "pos_x": -31.5,
+ "pos_y": 170.34375,
+ "pos_z": -16.6875,
+ "stations": "O'Donnell Orbital,Naddoddur Orbital,Anderson Landing"
+ },
+ {
+ "name": "Kamocan",
+ "pos_x": 74.40625,
+ "pos_y": -115.90625,
+ "pos_z": 89.65625,
+ "stations": "Greene Dock,Danforth City,Leonard Orbital,Charlier City,Merril Bastion,Doyle Point,Roggeveen Base,Potocnik Hub,Leckie Lab,Gasparis Vision,Littrow Gateway,Kemurdzhian Installation,Miller Gateway,Palisa Orbital,Pajdusakova Terminal"
+ },
+ {
+ "name": "Kamorin",
+ "pos_x": -123.875,
+ "pos_y": -81.59375,
+ "pos_z": 45.1875,
+ "stations": "Godwin Vision,Sterling Settlement"
+ },
+ {
+ "name": "Kamsety",
+ "pos_x": 110.375,
+ "pos_y": -144.25,
+ "pos_z": 113.53125,
+ "stations": "Sternbach Silo,Bond Station,Mukai Orbital,Axon Orbital,Messerschmitt Orbital,Extra Landing,Gorgani Terminal,Alfven Landing,Balmer Base"
+ },
+ {
+ "name": "Kamu Yamato",
+ "pos_x": 35.75,
+ "pos_y": 23.90625,
+ "pos_z": -144.21875,
+ "stations": "Sherrington Enterprise,Comer Installation,Thoreau Dock"
+ },
+ {
+ "name": "Kamumba",
+ "pos_x": 154.53125,
+ "pos_y": -67.21875,
+ "pos_z": 29.5625,
+ "stations": "Truman Station,Deslandres Landing,Molchanov Reformatory,Kepler Base"
+ },
+ {
+ "name": "Kamunkula",
+ "pos_x": 162.09375,
+ "pos_y": -118.0625,
+ "pos_z": 25.71875,
+ "stations": "Dornier Landing"
+ },
+ {
+ "name": "Kamur",
+ "pos_x": 145.53125,
+ "pos_y": 61.625,
+ "pos_z": -78.53125,
+ "stations": "Aitken Vision,Dashiell Terminal"
+ },
+ {
+ "name": "Kamuti",
+ "pos_x": -38.5625,
+ "pos_y": 27.65625,
+ "pos_z": -104.53125,
+ "stations": "Holden's Inheritance,Huygens Terminal,Smith Terminal,Chretien Orbital,Stairs' Progress"
+ },
+ {
+ "name": "Kamuy",
+ "pos_x": 18.625,
+ "pos_y": 10.78125,
+ "pos_z": 45.1875,
+ "stations": "Low Bastion,Ockels Terminal"
+ },
+ {
+ "name": "Kan Ajana",
+ "pos_x": 166.96875,
+ "pos_y": -60.15625,
+ "pos_z": -23.71875,
+ "stations": "Young Landing,Barmin Point,Russell Mines"
+ },
+ {
+ "name": "Kan Aphu",
+ "pos_x": 20.375,
+ "pos_y": 120.8125,
+ "pos_z": 21.96875,
+ "stations": "Daley Platform,Tennyson d'Eyncourt Barracks"
+ },
+ {
+ "name": "Kan Guru",
+ "pos_x": -120.4375,
+ "pos_y": -17.1875,
+ "pos_z": -42.5625,
+ "stations": "Varley Mines"
+ },
+ {
+ "name": "Kan Nu",
+ "pos_x": 152.9375,
+ "pos_y": 6.96875,
+ "pos_z": -32.71875,
+ "stations": "Kovalevsky Port,Melnick Terminal,Baker Installation"
+ },
+ {
+ "name": "Kan Zang",
+ "pos_x": 120.90625,
+ "pos_y": 58.59375,
+ "pos_z": 42.9375,
+ "stations": "Oltion Dock"
+ },
+ {
+ "name": "Kana",
+ "pos_x": 54.71875,
+ "pos_y": -140.15625,
+ "pos_z": 102.09375,
+ "stations": "Penzias Vision,Friedman Mines"
+ },
+ {
+ "name": "Kanabilamai",
+ "pos_x": 120.25,
+ "pos_y": 31.125,
+ "pos_z": 17.03125,
+ "stations": "Garay Platform,Goddard Orbital"
+ },
+ {
+ "name": "Kanagoto",
+ "pos_x": 155.46875,
+ "pos_y": -109.59375,
+ "pos_z": -4.15625,
+ "stations": "Kemurdzhian Enterprise,Truman's Progress,Struve Hub,Penrose Works,Wilhelm Klinkerfues Dock"
+ },
+ {
+ "name": "Kanaheim",
+ "pos_x": -37.40625,
+ "pos_y": -99.40625,
+ "pos_z": 36.65625,
+ "stations": "Weizsacker Settlement,Eskridge Landing,Sutter Dock,Hawker Hub"
+ },
+ {
+ "name": "Kanaloa",
+ "pos_x": 75.09375,
+ "pos_y": -39.34375,
+ "pos_z": -24.0625,
+ "stations": "Allen Terminal,Koontz's Inheritance,Linnehan Terminal,Poisson Laboratory,Penn Depot"
+ },
+ {
+ "name": "Kanamba",
+ "pos_x": 103.4375,
+ "pos_y": 10.9375,
+ "pos_z": -56.84375,
+ "stations": "Vaucanson Depot"
+ },
+ {
+ "name": "Kanandos",
+ "pos_x": 84.28125,
+ "pos_y": -45.0625,
+ "pos_z": -62.0625,
+ "stations": "Ricardo Installation,Anderson City,Wylie Penitentiary"
+ },
+ {
+ "name": "Kanates",
+ "pos_x": 105.65625,
+ "pos_y": -65.03125,
+ "pos_z": -18.0625,
+ "stations": "Otus Port,Yano Landing"
+ },
+ {
+ "name": "Kanati",
+ "pos_x": 74.125,
+ "pos_y": 46.1875,
+ "pos_z": -19.46875,
+ "stations": "Lucid Dock,Yegorov Gateway"
+ },
+ {
+ "name": "Kanawas",
+ "pos_x": 62.1875,
+ "pos_y": -94,
+ "pos_z": -30.25,
+ "stations": "Shoujing Terminal,Harvia Terminal,Kondo Landing"
+ },
+ {
+ "name": "Kandama",
+ "pos_x": -3.6875,
+ "pos_y": 19.28125,
+ "pos_z": 117.1875,
+ "stations": "Clark Dock,Diophantus Beacon,Parry Beacon,Ziljak Silo"
+ },
+ {
+ "name": "Kandanda",
+ "pos_x": -53.34375,
+ "pos_y": 98.34375,
+ "pos_z": 87.03125,
+ "stations": "Zhigang Point,Siemens Outpost"
+ },
+ {
+ "name": "Kandha",
+ "pos_x": -76.875,
+ "pos_y": -80.375,
+ "pos_z": 74.53125,
+ "stations": "Rennie's Progress,Bhabha Platform"
+ },
+ {
+ "name": "Kandiil",
+ "pos_x": -1.15625,
+ "pos_y": 123.28125,
+ "pos_z": 35.25,
+ "stations": "Slonczewski Prospect,Mattingly Hub,Morin Hub,Giles Beacon,Bayliss Enterprise"
+ },
+ {
+ "name": "Kandit",
+ "pos_x": 37.5625,
+ "pos_y": 159.46875,
+ "pos_z": 22.5625,
+ "stations": "Tshang Outpost"
+ },
+ {
+ "name": "Kandji",
+ "pos_x": 169.40625,
+ "pos_y": -59.0625,
+ "pos_z": 64.8125,
+ "stations": "Hussenot Landing,Furuta Prospect,Gurney Dock"
+ },
+ {
+ "name": "Kanean",
+ "pos_x": 22.28125,
+ "pos_y": -22.75,
+ "pos_z": -86.46875,
+ "stations": "Malyshev Horizons,Duke Port,Melvin Relay,Wiley Terminal,Huberath Horizons"
+ },
+ {
+ "name": "Kanga Bara",
+ "pos_x": -58.40625,
+ "pos_y": 121.28125,
+ "pos_z": -116.71875,
+ "stations": "Schottky Survey"
+ },
+ {
+ "name": "Kangthitani",
+ "pos_x": 15.90625,
+ "pos_y": -158.84375,
+ "pos_z": 161.375,
+ "stations": "Hirasawa Retreat,Leckie Prospect,Israel Retreat"
+ },
+ {
+ "name": "Kangulu",
+ "pos_x": 142.875,
+ "pos_y": -159.53125,
+ "pos_z": 104.5625,
+ "stations": "Barlowe Hub,Barbuy Port,Wegner Arsenal,Hollander Vision"
+ },
+ {
+ "name": "Kanians",
+ "pos_x": 44.84375,
+ "pos_y": 98.09375,
+ "pos_z": 55.0625,
+ "stations": "Ballard Market,Younghusband Orbital,al-Khowarizmi Terminal,Dummer Dock,Reamy Port,Forsskal Camp,Palmer Terminal,White Terminal,Bentham Terminal,Boulle Installation,Franke Relay,Rawn Holdings"
+ },
+ {
+ "name": "Kanitisa",
+ "pos_x": 23.03125,
+ "pos_y": -118.5,
+ "pos_z": 42.75,
+ "stations": "Witt Ring,Dubyago Dock,Adkins Dock,Cummings Landing"
+ },
+ {
+ "name": "Kaniya",
+ "pos_x": 163.21875,
+ "pos_y": -100.75,
+ "pos_z": 56.03125,
+ "stations": "Hickam Vision,MacCready Prospect,Hilbert Beacon"
+ },
+ {
+ "name": "Kannari",
+ "pos_x": 85.9375,
+ "pos_y": 10.03125,
+ "pos_z": -108.9375,
+ "stations": "Culbertson Terminal,Shonin Depot,Lessing Relay"
+ },
+ {
+ "name": "Kannicas",
+ "pos_x": -0.21875,
+ "pos_y": 81.1875,
+ "pos_z": 66.59375,
+ "stations": "Friend Terminal,Pullman City,Morrison Orbital,Ostrander Forum,Effinger Holdings"
+ },
+ {
+ "name": "Kannon",
+ "pos_x": -72.71875,
+ "pos_y": -122.84375,
+ "pos_z": 8.5,
+ "stations": "Ross City"
+ },
+ {
+ "name": "Kannona",
+ "pos_x": 101.3125,
+ "pos_y": 99.78125,
+ "pos_z": 86.5625,
+ "stations": "Runco Landing"
+ },
+ {
+ "name": "Kano",
+ "pos_x": 168.1875,
+ "pos_y": -97.5625,
+ "pos_z": 120.0625,
+ "stations": "Verrier Survey,Goryu Refinery,Stevenson Mines,Smith Prospect,Jewitt Port"
+ },
+ {
+ "name": "Kanoai",
+ "pos_x": 63.40625,
+ "pos_y": -206,
+ "pos_z": 104.5,
+ "stations": "McManus Enterprise,Argelander Terminal"
+ },
+ {
+ "name": "Kanomota",
+ "pos_x": -41.53125,
+ "pos_y": -143.21875,
+ "pos_z": -12.8125,
+ "stations": "Bouwens Port,Fairbairn Holdings,Maunder Orbital"
+ },
+ {
+ "name": "Kanooks",
+ "pos_x": -18.09375,
+ "pos_y": 82.5,
+ "pos_z": 140.21875,
+ "stations": "Drexler Port,Edison Hub,Banks Platform,Klimuk Legacy,McIntyre Keep"
+ },
+ {
+ "name": "Kanopi",
+ "pos_x": 59.96875,
+ "pos_y": 2.6875,
+ "pos_z": -107.625,
+ "stations": "Kimbrough Mines"
+ },
+ {
+ "name": "Kanos",
+ "pos_x": 41.375,
+ "pos_y": 53.65625,
+ "pos_z": -12.65625,
+ "stations": "Geston Ring,Lewitt Camp,Grego Arsenal"
+ },
+ {
+ "name": "Kansei",
+ "pos_x": -35.28125,
+ "pos_y": 96.84375,
+ "pos_z": 74.46875,
+ "stations": "Burnell Beacon"
+ },
+ {
+ "name": "Kanu",
+ "pos_x": 113.4375,
+ "pos_y": -23.03125,
+ "pos_z": -124.28125,
+ "stations": "Beaumont Survey"
+ },
+ {
+ "name": "Kanuket",
+ "pos_x": 57.3125,
+ "pos_y": -163.28125,
+ "pos_z": 88,
+ "stations": "Flaugergues Gateway,Mueller Port,Pilcher Enterprise,Chongzhi Hub"
+ },
+ {
+ "name": "Kanur",
+ "pos_x": -22.125,
+ "pos_y": -24.90625,
+ "pos_z": 96.96875,
+ "stations": "Pierres Relay,Swift Gateway,Nagata City,Lopez de Legazpi Laboratory"
+ },
+ {
+ "name": "Kanus",
+ "pos_x": -97.125,
+ "pos_y": 41.375,
+ "pos_z": -71.71875,
+ "stations": "Oefelein Refinery,Sommerfeld Camp"
+ },
+ {
+ "name": "Kanzicnal",
+ "pos_x": 39.34375,
+ "pos_y": -179.3125,
+ "pos_z": 38.40625,
+ "stations": "Theodor Winnecke Gateway,Littrow Ring,Michell Dock,Barentsz Laboratory,Finlay Survey"
+ },
+ {
+ "name": "Kao Guojiu",
+ "pos_x": 109.96875,
+ "pos_y": -186.0625,
+ "pos_z": -15.84375,
+ "stations": "Leydenfrost Settlement,Stiegler Arena,Maupertuis Dock"
+ },
+ {
+ "name": "Kao Jundji",
+ "pos_x": 111.8125,
+ "pos_y": -193.1875,
+ "pos_z": 97.125,
+ "stations": "Pickering Mines,Lundmark Relay,Turner's Inheritance,Morris Landing"
+ },
+ {
+ "name": "Kao Kach",
+ "pos_x": -73.5625,
+ "pos_y": -49.78125,
+ "pos_z": 35.59375,
+ "stations": "Meikle Landing,Betancourt Works,Dowling Depot"
+ },
+ {
+ "name": "Kao Thewi",
+ "pos_x": 99.5625,
+ "pos_y": -64.75,
+ "pos_z": 0.03125,
+ "stations": "Geston Landing,Rodrigues Bastion,Alvarez de Pineda Settlement"
+ },
+ {
+ "name": "Kao Wanga",
+ "pos_x": 114.21875,
+ "pos_y": -75.40625,
+ "pos_z": 72.90625,
+ "stations": "De City,Sorayama Estate,O'Brien Point,Bond Gateway,de Kamp Terminal"
+ },
+ {
+ "name": "Kao Ziyi",
+ "pos_x": 58.15625,
+ "pos_y": 1.78125,
+ "pos_z": -71.8125,
+ "stations": "Gillekens Hub,Hedley Vision,Peirce Reformatory"
+ },
+ {
+ "name": "Kapod",
+ "pos_x": 59.53125,
+ "pos_y": -83.53125,
+ "pos_z": -23.8125,
+ "stations": "Emshwiller Orbital,Berkey Terminal,Michalitsianos Hub,Alexander Mine,Kraft Point"
+ },
+ {
+ "name": "Kapoongzi",
+ "pos_x": 115.09375,
+ "pos_y": -122.625,
+ "pos_z": 119.03125,
+ "stations": "Jones Hub,Herbig Base,Liouville Vision"
+ },
+ {
+ "name": "Kappa",
+ "pos_x": 120.96875,
+ "pos_y": -11.90625,
+ "pos_z": -85.15625,
+ "stations": "Poisson Orbital,Darboux Station,Nielsen Depot,Bertin Ring,Younghusband Beacon"
+ },
+ {
+ "name": "Kappa Andromedae",
+ "pos_x": -151.40625,
+ "pos_y": -48.03125,
+ "pos_z": -55.96875,
+ "stations": "Broglie Platform,Muhammad Ibn Battuta Survey,Yegorov Colony"
+ },
+ {
+ "name": "Kappa Doradus",
+ "pos_x": 169.90625,
+ "pos_y": -137.53125,
+ "pos_z": -2.09375,
+ "stations": "Schwarzschild Port,Goodricke Dock,Arkhangelsky Port"
+ },
+ {
+ "name": "Kappa Fornacis",
+ "pos_x": 12.46875,
+ "pos_y": -66.71875,
+ "pos_z": -22.90625,
+ "stations": "Harvestport,Shriver Hub,Tshang Enterprise,Mukai's Folly"
+ },
+ {
+ "name": "Kappa Ophiuchi",
+ "pos_x": -38.3125,
+ "pos_y": 44.25,
+ "pos_z": 70.28125,
+ "stations": "Koch Dock,Peterson Terminal,Cockrell City,Blodgett Gateway,Stephenson City,Marley Base,Vries Gateway,Boming Installation,McCarthy Depot"
+ },
+ {
+ "name": "Kappa Phoenicis",
+ "pos_x": 15.3125,
+ "pos_y": -74.3125,
+ "pos_z": 16.59375,
+ "stations": "Filipchenko Hub,Gresley Landing,Low Gateway"
+ },
+ {
+ "name": "Kappa Reticuli",
+ "pos_x": 48.65625,
+ "pos_y": -50.84375,
+ "pos_z": 6.875,
+ "stations": "Newman Hub,Smeaton Hub,Ivanchenkov Settlement,Schirra Dock,Celsius Terminal,Roosa Dock,Bobko Gateway,Rose Settlement,Thompson Depot,Conway Installation"
+ },
+ {
+ "name": "Kappa Tucanae",
+ "pos_x": 39.5625,
+ "pos_y": -51.0625,
+ "pos_z": 22.28125,
+ "stations": "Ortiz Moreno City,Faber Terminal,Skiff Port,Gent Hub,Hollander Base,Agrippa Enterprise"
+ },
+ {
+ "name": "Kappa-1 Ceti",
+ "pos_x": -0.53125,
+ "pos_y": -20.15625,
+ "pos_z": -22,
+ "stations": "Konscak Silo,Kroehl Platform,Taine Colony"
+ },
+ {
+ "name": "Kappa-1 Coronae Austrinae",
+ "pos_x": 9.59375,
+ "pos_y": -37.09375,
+ "pos_z": 149.15625,
+ "stations": "Matthews Beacon,Barnes Bastion"
+ },
+ {
+ "name": "Kappanii",
+ "pos_x": 30.09375,
+ "pos_y": 66.625,
+ "pos_z": -81.125,
+ "stations": "Halley Port,Szameit Enterprise"
+ },
+ {
+ "name": "Kapperapai",
+ "pos_x": -115.03125,
+ "pos_y": 2.03125,
+ "pos_z": 86.71875,
+ "stations": "Weitz Landing,Shiner Keep"
+ },
+ {
+ "name": "Kapura",
+ "pos_x": 135.25,
+ "pos_y": 35.78125,
+ "pos_z": -44.8125,
+ "stations": "McDonald Horizons"
+ },
+ {
+ "name": "Kapurri",
+ "pos_x": 49.53125,
+ "pos_y": -125.34375,
+ "pos_z": 75.21875,
+ "stations": "Tan Hub,Rosse Dock,Curtis Colony,Vardeman's Folly,Jewitt Horizons"
+ },
+ {
+ "name": "Kapus",
+ "pos_x": -146.53125,
+ "pos_y": 59.3125,
+ "pos_z": 63.40625,
+ "stations": "Gaspar de Lemos' Exile"
+ },
+ {
+ "name": "Kaqchina",
+ "pos_x": 120.1875,
+ "pos_y": 13.28125,
+ "pos_z": -74.3125,
+ "stations": "Dalton Beacon,Garneau Landing"
+ },
+ {
+ "name": "Kaqchis",
+ "pos_x": -36.03125,
+ "pos_y": -154.5625,
+ "pos_z": -8.59375,
+ "stations": "Abetti Colony,Gamow Colony,Fancher's Claim"
+ },
+ {
+ "name": "Kara",
+ "pos_x": -29.75,
+ "pos_y": -58.8125,
+ "pos_z": -163.15625,
+ "stations": "Garn Point"
+ },
+ {
+ "name": "Karabal",
+ "pos_x": -8.40625,
+ "pos_y": -99.9375,
+ "pos_z": -39.28125,
+ "stations": "Nusslein-Volhard Hub,Hutchinson Base,Jett Hub,Weston Enterprise,Perrin Orbital"
+ },
+ {
+ "name": "Karaces",
+ "pos_x": -98.34375,
+ "pos_y": 36.875,
+ "pos_z": 96.5,
+ "stations": "Morgan Dock"
+ },
+ {
+ "name": "Karadjari",
+ "pos_x": 60.875,
+ "pos_y": -115.40625,
+ "pos_z": 38.09375,
+ "stations": "Dufay Vision,Lemaitre Prospect,Seares Mines,Millosevich's Progress"
+ },
+ {
+ "name": "Karagro",
+ "pos_x": -4.3125,
+ "pos_y": 33.1875,
+ "pos_z": 70.125,
+ "stations": "Snyder City,Sullivan Colony,Hopkins Port,Fontenay Vision,Gibson Relay"
+ },
+ {
+ "name": "Karaikda",
+ "pos_x": 133.71875,
+ "pos_y": 0.09375,
+ "pos_z": 82.75,
+ "stations": "Leeuwenhoek Port,Wundt Port,Leoniceno Mines"
+ },
+ {
+ "name": "Karakamane",
+ "pos_x": 73.34375,
+ "pos_y": -184.40625,
+ "pos_z": 84.4375,
+ "stations": "Hirasawa Point,Bainbridge's Pride,Fernandes Terminal"
+ },
+ {
+ "name": "Karakasis",
+ "pos_x": 124.53125,
+ "pos_y": -186.21875,
+ "pos_z": 33.15625,
+ "stations": "Ziegler Orbital,Hussey Colony,Resnik Vision,Nansen Depot,Auwers Depot"
+ },
+ {
+ "name": "Karakmo",
+ "pos_x": 169.84375,
+ "pos_y": -118.90625,
+ "pos_z": -9.90625,
+ "stations": "Pippin Landing,Harawi Survey,Vyssotsky Prospect"
+ },
+ {
+ "name": "Karama",
+ "pos_x": 72.34375,
+ "pos_y": -103.5,
+ "pos_z": 67.875,
+ "stations": "Marth Port,i Sola Ring,Kotzebue Barracks,Miller Enterprise"
+ },
+ {
+ "name": "Karamai",
+ "pos_x": 142.71875,
+ "pos_y": 1.96875,
+ "pos_z": 39.25,
+ "stations": "Hulse Orbital,Dilworth Hub,Nouvel Survey,Ryan Point,Blaylock Beacon"
+ },
+ {
+ "name": "Karamayayak",
+ "pos_x": -32.6875,
+ "pos_y": -62.8125,
+ "pos_z": 130.09375,
+ "stations": "Shiras Port"
+ },
+ {
+ "name": "Karamo",
+ "pos_x": 108.09375,
+ "pos_y": 17,
+ "pos_z": 100.1875,
+ "stations": "Karlsefni Bastion,Einstein Settlement,Mukai Camp"
+ },
+ {
+ "name": "Karamojola",
+ "pos_x": 133.4375,
+ "pos_y": -126.40625,
+ "pos_z": 79.6875,
+ "stations": "Frost Horizons,Truman Platform"
+ },
+ {
+ "name": "Karamu",
+ "pos_x": -16.71875,
+ "pos_y": 123.3125,
+ "pos_z": -110.15625,
+ "stations": "Barjavel Works,Veblen Dock,Dummer Vision"
+ },
+ {
+ "name": "Karanguru",
+ "pos_x": 178.15625,
+ "pos_y": -133.28125,
+ "pos_z": 44.5625,
+ "stations": "Cavendish Settlement,Dumont Terminal,Cartier Platform,Schoenherr Platform"
+ },
+ {
+ "name": "Karas",
+ "pos_x": -160.8125,
+ "pos_y": -33.21875,
+ "pos_z": 9.3125,
+ "stations": "Wood Refinery"
+ },
+ {
+ "name": "Karasiginn",
+ "pos_x": -64.28125,
+ "pos_y": -98.125,
+ "pos_z": 78.90625,
+ "stations": "Galvani Mines"
+ },
+ {
+ "name": "Karasiri",
+ "pos_x": -91.1875,
+ "pos_y": -141.4375,
+ "pos_z": 56.34375,
+ "stations": "Fozard Platform,Pellegrino Vision"
+ },
+ {
+ "name": "Karasvara",
+ "pos_x": 49.25,
+ "pos_y": -118.0625,
+ "pos_z": 119.65625,
+ "stations": "Suydam City,Medupe Station,Phillips Port,Schomburg Vision,Schmidt City,Reed Depot"
+ },
+ {
+ "name": "Karatayo",
+ "pos_x": -77.8125,
+ "pos_y": 48.1875,
+ "pos_z": 116,
+ "stations": "Makeev Oasis,Cogswell Oasis,Herreshoff Terminal"
+ },
+ {
+ "name": "Karbacoc",
+ "pos_x": 112.65625,
+ "pos_y": -25.875,
+ "pos_z": -96.0625,
+ "stations": "Hewish Enterprise,Selye Dock"
+ },
+ {
+ "name": "Karbon",
+ "pos_x": -93.125,
+ "pos_y": 102.8125,
+ "pos_z": -114.75,
+ "stations": "Budrys Installation,Spring Refinery,Euthymenes Prospect,Brin Colony,Bogdanov's Progress"
+ },
+ {
+ "name": "Karbudj",
+ "pos_x": 80.1875,
+ "pos_y": -84.875,
+ "pos_z": 100.25,
+ "stations": "Shirazi Station"
+ },
+ {
+ "name": "Karbudji",
+ "pos_x": -118.125,
+ "pos_y": 60.6875,
+ "pos_z": -34.6875,
+ "stations": "Fisk Colony,Greenland Dock,Slonczewski Settlement,Waldrop Base"
+ },
+ {
+ "name": "Kareco",
+ "pos_x": 20.8125,
+ "pos_y": -109.28125,
+ "pos_z": -22.75,
+ "stations": "Brill Station,Martin Vista"
+ },
+ {
+ "name": "Karecoya",
+ "pos_x": 96.28125,
+ "pos_y": 0.84375,
+ "pos_z": 47.9375,
+ "stations": "Linnaeus Platform"
+ },
+ {
+ "name": "Kared",
+ "pos_x": 45.34375,
+ "pos_y": -35.21875,
+ "pos_z": -81.125,
+ "stations": "Stuart Station,McMahon Reach,Iben Installation"
+ },
+ {
+ "name": "Kareldt",
+ "pos_x": -95.6875,
+ "pos_y": -95.59375,
+ "pos_z": 109,
+ "stations": "Bondar Gateway,Fairbairn Enterprise,Berliner Terminal,Griffith Silo,Wilder Landing"
+ },
+ {
+ "name": "Karelitakas",
+ "pos_x": 24.0625,
+ "pos_y": -143.9375,
+ "pos_z": -19.53125,
+ "stations": "Glass Station,Cartier Hub"
+ },
+ {
+ "name": "Karen",
+ "pos_x": -30.03125,
+ "pos_y": 6.5,
+ "pos_z": 98,
+ "stations": "Morin Vista,Crown Outpost"
+ },
+ {
+ "name": "Kareneri",
+ "pos_x": -19.03125,
+ "pos_y": 2.5,
+ "pos_z": -74.28125,
+ "stations": "Elion Terminal"
+ },
+ {
+ "name": "Karengi",
+ "pos_x": 68.25,
+ "pos_y": 61.25,
+ "pos_z": -86.78125,
+ "stations": "Burton Port"
+ },
+ {
+ "name": "Karenses",
+ "pos_x": -125.375,
+ "pos_y": 2.59375,
+ "pos_z": -73.96875,
+ "stations": "Dedekind Colony"
+ },
+ {
+ "name": "Kareri",
+ "pos_x": -56.625,
+ "pos_y": 19.59375,
+ "pos_z": 111.4375,
+ "stations": "Gooch Settlement"
+ },
+ {
+ "name": "Karetii",
+ "pos_x": -125.59375,
+ "pos_y": 44.03125,
+ "pos_z": 78.40625,
+ "stations": "Bridges Settlement,Appel Horizons,Sinclair Platform,Soper Silo,Ampere Horizons"
+ },
+ {
+ "name": "Karex",
+ "pos_x": -99.28125,
+ "pos_y": -108.15625,
+ "pos_z": 35.21875,
+ "stations": "Ross Settlement,Zudov Colony"
+ },
+ {
+ "name": "Kariara",
+ "pos_x": -2.875,
+ "pos_y": -180.3125,
+ "pos_z": 30.96875,
+ "stations": "Elson Works"
+ },
+ {
+ "name": "Karid",
+ "pos_x": 85.0625,
+ "pos_y": -91.125,
+ "pos_z": 58.90625,
+ "stations": "Araki Port,Kobayashi Gateway,Friend Settlement,Condit Hub"
+ },
+ {
+ "name": "Karini",
+ "pos_x": -93.4375,
+ "pos_y": -1.9375,
+ "pos_z": -3,
+ "stations": "Lu Relay,Fulton Dock"
+ },
+ {
+ "name": "Karis",
+ "pos_x": 64.46875,
+ "pos_y": 32.15625,
+ "pos_z": -46.59375,
+ "stations": "Glazkov Orbital,Haignere Mines,Rawat Base,Avogadro Vision,Lawhead Installation"
+ },
+ {
+ "name": "Karishnu",
+ "pos_x": 0.3125,
+ "pos_y": -16.0625,
+ "pos_z": -127.96875,
+ "stations": "Wright Refinery,Nolan Depot"
+ },
+ {
+ "name": "Karitis",
+ "pos_x": -78.75,
+ "pos_y": 81.09375,
+ "pos_z": 69.40625,
+ "stations": "Grijalva Dock,de Balboa Survey"
+ },
+ {
+ "name": "Karka",
+ "pos_x": 134.125,
+ "pos_y": 15.09375,
+ "pos_z": -63.875,
+ "stations": "Stith Enterprise,Chandler Ring,Gresh Terminal"
+ },
+ {
+ "name": "Karkates",
+ "pos_x": -70.21875,
+ "pos_y": 96.375,
+ "pos_z": 116.96875,
+ "stations": "Jiushao Settlement,Rowley Mines,Boole Installation"
+ },
+ {
+ "name": "Karkinicha",
+ "pos_x": 109.0625,
+ "pos_y": 76.90625,
+ "pos_z": 20.28125,
+ "stations": "Aldiss Survey,Loncke Keep,Pordenone Survey"
+ },
+ {
+ "name": "Karm",
+ "pos_x": 16.03125,
+ "pos_y": -247.8125,
+ "pos_z": 47.84375,
+ "stations": "Ashbrook Oasis,Lynden-Bell Settlement,Smoot Keep"
+ },
+ {
+ "name": "Karna",
+ "pos_x": 147.78125,
+ "pos_y": -162.875,
+ "pos_z": 111.5625,
+ "stations": "Wang Terminal"
+ },
+ {
+ "name": "Karnae",
+ "pos_x": -51.3125,
+ "pos_y": -122.8125,
+ "pos_z": -3.40625,
+ "stations": "Paulmier de Gonneville Installation,Dedman Mines,Miklouho-Maclay Platform"
+ },
+ {
+ "name": "Karnarki",
+ "pos_x": 77.4375,
+ "pos_y": -1.375,
+ "pos_z": 13.78125,
+ "stations": "Haignere Enterprise,Pangborn City,Marusek Orbital"
+ },
+ {
+ "name": "Karnir",
+ "pos_x": 133.03125,
+ "pos_y": -23.5,
+ "pos_z": 113.625,
+ "stations": "Nobel Outpost"
+ },
+ {
+ "name": "Karo",
+ "pos_x": 131.34375,
+ "pos_y": -12.59375,
+ "pos_z": 0.21875,
+ "stations": "Escobar Dock"
+ },
+ {
+ "name": "Karogonibo",
+ "pos_x": -118.5,
+ "pos_y": -30.21875,
+ "pos_z": 65.84375,
+ "stations": "Gantt Terminal,Cortes Laboratory"
+ },
+ {
+ "name": "Karourok",
+ "pos_x": -65.03125,
+ "pos_y": 6.15625,
+ "pos_z": -143.15625,
+ "stations": "Forstchen Camp,Narbeth Stop"
+ },
+ {
+ "name": "Karovices",
+ "pos_x": -102.4375,
+ "pos_y": 6.9375,
+ "pos_z": -14.875,
+ "stations": "Hurley Terminal,Brandenstein Platform"
+ },
+ {
+ "name": "Karovoy",
+ "pos_x": -38.8125,
+ "pos_y": -74.53125,
+ "pos_z": 51.75,
+ "stations": "Sellings Landing,Rondon Freeport,Shatner Dock"
+ },
+ {
+ "name": "Karsha",
+ "pos_x": 54.5,
+ "pos_y": -79.46875,
+ "pos_z": 58.59375,
+ "stations": "Napier Depot,Beg Platform"
+ },
+ {
+ "name": "Karsinanari",
+ "pos_x": -29.875,
+ "pos_y": -46.1875,
+ "pos_z": -8.03125,
+ "stations": "Sucharitkul Hub,Shalatula Terminal,Nasmyth Mines"
+ },
+ {
+ "name": "Karsing",
+ "pos_x": 29.15625,
+ "pos_y": -20.9375,
+ "pos_z": -120.59375,
+ "stations": "Lister Horizons"
+ },
+ {
+ "name": "Karsu",
+ "pos_x": -84.03125,
+ "pos_y": -42.96875,
+ "pos_z": -75.75,
+ "stations": "Kondratyev Prospect,Sternberg Point"
+ },
+ {
+ "name": "Karsuki Ti",
+ "pos_x": 134.03125,
+ "pos_y": -163.59375,
+ "pos_z": 71.0625,
+ "stations": "West Market,Ikeya Vision,Helin Gateway,Wilson Gateway,Koolhaas Hub,Blaauw Dock,Shelley Installation,Malvern Landing"
+ },
+ {
+ "name": "Kartam",
+ "pos_x": -34.6875,
+ "pos_y": -75.03125,
+ "pos_z": 49.75,
+ "stations": "Teller Landing,Rasmussen Prospect,Wohler Platform"
+ },
+ {
+ "name": "Kartamayana",
+ "pos_x": 57.0625,
+ "pos_y": -143.25,
+ "pos_z": 18,
+ "stations": "Hayashi Installation,McManus Survey,Slipher Survey"
+ },
+ {
+ "name": "Kartamoan",
+ "pos_x": 146.59375,
+ "pos_y": -159.84375,
+ "pos_z": 114.40625,
+ "stations": "Becvar Works"
+ },
+ {
+ "name": "Karten",
+ "pos_x": 15.15625,
+ "pos_y": 94.5,
+ "pos_z": -39.3125,
+ "stations": "Robinson Mines,Coulter Settlement"
+ },
+ {
+ "name": "Kartenes",
+ "pos_x": 161.5,
+ "pos_y": -104,
+ "pos_z": -17.21875,
+ "stations": "Parkinson Port,Engle Hub,Dawes Orbital"
+ },
+ {
+ "name": "Kartudjara",
+ "pos_x": 82.28125,
+ "pos_y": 73,
+ "pos_z": -117.53125,
+ "stations": "Chomsky Mine,Smith Camp"
+ },
+ {
+ "name": "Karuekenus",
+ "pos_x": -37.3125,
+ "pos_y": 35.28125,
+ "pos_z": 159.6875,
+ "stations": "Staden Landing,Gotlieb Settlement,Baudin's Folly"
+ },
+ {
+ "name": "Karugbara",
+ "pos_x": -19.65625,
+ "pos_y": 139.09375,
+ "pos_z": -51.4375,
+ "stations": "Weil Beacon"
+ },
+ {
+ "name": "Karunti",
+ "pos_x": 132.5625,
+ "pos_y": -240.53125,
+ "pos_z": 115.5,
+ "stations": "Barnwell Prospect,Elmore's Claim,Reynolds Installation"
+ },
+ {
+ "name": "Karwareti",
+ "pos_x": 18.25,
+ "pos_y": -199.625,
+ "pos_z": -20.625,
+ "stations": "Mil's Inheritance,Cantor Horizons"
+ },
+ {
+ "name": "KashiGaut",
+ "pos_x": 50.25,
+ "pos_y": 106.3125,
+ "pos_z": -89.21875,
+ "stations": "Sevastyanov Settlement,Reisman Platform"
+ },
+ {
+ "name": "Kashis",
+ "pos_x": -160.0625,
+ "pos_y": 10.03125,
+ "pos_z": -65.84375,
+ "stations": "Soto Reach,Boulle Mines"
+ },
+ {
+ "name": "Kasho",
+ "pos_x": -19.40625,
+ "pos_y": 24.59375,
+ "pos_z": -172.5625,
+ "stations": "Sagan Dock,Payne Terminal,Semeonis Port,Serling Base"
+ },
+ {
+ "name": "Kashyapa",
+ "pos_x": -8392.03125,
+ "pos_y": -701.03125,
+ "pos_z": 17555.125,
+ "stations": "Vihara Gate,Martyrs' Rest"
+ },
+ {
+ "name": "Kassi",
+ "pos_x": 36.25,
+ "pos_y": 136.0625,
+ "pos_z": -97.59375,
+ "stations": "Carver Horizons,Butler Base,Taine Station,Hunt Terminal,Bailey Terminal"
+ },
+ {
+ "name": "Kassimshipa",
+ "pos_x": -9.34375,
+ "pos_y": -111.65625,
+ "pos_z": -32.5625,
+ "stations": "Aleksandrov Vision,Nicollet Relay,Ockels Terminal"
+ },
+ {
+ "name": "Kasszony",
+ "pos_x": -85.125,
+ "pos_y": -43.25,
+ "pos_z": 82.40625,
+ "stations": "Culpeper Plant"
+ },
+ {
+ "name": "Kasya",
+ "pos_x": 43.09375,
+ "pos_y": 27.34375,
+ "pos_z": 107.625,
+ "stations": "Williams Horizons"
+ },
+ {
+ "name": "Kata",
+ "pos_x": -35.375,
+ "pos_y": 70.03125,
+ "pos_z": 130.9375,
+ "stations": "Dobrovolskiy Colony,Wankel Penal colony"
+ },
+ {
+ "name": "Kataning",
+ "pos_x": 56.21875,
+ "pos_y": -86.78125,
+ "pos_z": 8.84375,
+ "stations": "Naubakht Port,Monge Penal colony,Dalgarno Hangar"
+ },
+ {
+ "name": "Katas",
+ "pos_x": 168.375,
+ "pos_y": -123.25,
+ "pos_z": -49.4375,
+ "stations": "Goldreich Vision,Michalitsianos Colony,Muhammad Ibn Battuta Relay"
+ },
+ {
+ "name": "Kathaima",
+ "pos_x": -117.96875,
+ "pos_y": 9.6875,
+ "pos_z": 74.53125,
+ "stations": "Pike Landing"
+ },
+ {
+ "name": "Kathans",
+ "pos_x": 75.28125,
+ "pos_y": -26.3125,
+ "pos_z": 64.28125,
+ "stations": "Klein Orbital,Cantor Hub,Stevens Prospect,Houtman Penal colony"
+ },
+ {
+ "name": "Kato",
+ "pos_x": -181.03125,
+ "pos_y": 26.3125,
+ "pos_z": -20.78125,
+ "stations": "Walker Station,Antonio de Andrade Retreat"
+ },
+ {
+ "name": "Katobri",
+ "pos_x": -97.3125,
+ "pos_y": 50.125,
+ "pos_z": 55.09375,
+ "stations": "Hoften Port"
+ },
+ {
+ "name": "Katobriges",
+ "pos_x": 15.28125,
+ "pos_y": 121.0625,
+ "pos_z": -162.78125,
+ "stations": "Paulo da Gama Relay,Sleator Settlement,Powers Observatory"
+ },
+ {
+ "name": "Katocudatta",
+ "pos_x": 48.875,
+ "pos_y": -13.78125,
+ "pos_z": -83.375,
+ "stations": "Coles Horizons,Burke Hub,Vardeman Base,Kirk Beacon"
+ },
+ {
+ "name": "Katola",
+ "pos_x": -41.15625,
+ "pos_y": -150.28125,
+ "pos_z": -50.1875,
+ "stations": "Babcock Vision"
+ },
+ {
+ "name": "Katosus",
+ "pos_x": -93.03125,
+ "pos_y": 94.125,
+ "pos_z": 66.75,
+ "stations": "Shatner Ring"
+ },
+ {
+ "name": "Kats",
+ "pos_x": 25.875,
+ "pos_y": -238.9375,
+ "pos_z": 84.03125,
+ "stations": "Truman Hub,Herschel Landing,Poyser Landing,Thurston Terminal,Popper's Progress"
+ },
+ {
+ "name": "Katsur",
+ "pos_x": -120.96875,
+ "pos_y": -13.53125,
+ "pos_z": -76.21875,
+ "stations": "Baker Horizons,Dickson Base"
+ },
+ {
+ "name": "Katta",
+ "pos_x": 67.53125,
+ "pos_y": 25,
+ "pos_z": 24.625,
+ "stations": "Freud's Inheritance,Serre Point"
+ },
+ {
+ "name": "Kattvari",
+ "pos_x": 97.34375,
+ "pos_y": -61.3125,
+ "pos_z": 78.84375,
+ "stations": "Struzan Platform"
+ },
+ {
+ "name": "Katuci",
+ "pos_x": -49.5625,
+ "pos_y": -125.4375,
+ "pos_z": 21.125,
+ "stations": "Mitchell Vision,Archer Port,Cartmill Base,Huxley Prospect"
+ },
+ {
+ "name": "Katui",
+ "pos_x": -108.71875,
+ "pos_y": -74.34375,
+ "pos_z": -36.96875,
+ "stations": "Leclerc Station,Celsius' Inheritance,Arnarson Point,Remek Dock"
+ },
+ {
+ "name": "Katukilal",
+ "pos_x": -49.4375,
+ "pos_y": 158.0625,
+ "pos_z": -11.625,
+ "stations": "Gardner Ring,Wingrove Terminal,Isherwood Station,Nicollet Horizons,Brule Landing"
+ },
+ {
+ "name": "Katung",
+ "pos_x": 86.21875,
+ "pos_y": 76.5625,
+ "pos_z": 112.0625,
+ "stations": "Slayton Base"
+ },
+ {
+ "name": "Katungkates",
+ "pos_x": 82.3125,
+ "pos_y": 68.78125,
+ "pos_z": 3.8125,
+ "stations": "Fisher Station,Artsebarsky Plant,Gemar Terminal"
+ },
+ {
+ "name": "Katuri",
+ "pos_x": -19.6875,
+ "pos_y": -6.0625,
+ "pos_z": 81.5625,
+ "stations": "Kagan City,Bogdanov City,Rubruck Hub,Priest City,Shiras Port,Fidalgo City,Oltion City,Pook Hub,Fullerton Port,Stjepan Seljan Port,Cook Landing"
+ },
+ {
+ "name": "Katurru",
+ "pos_x": 17.625,
+ "pos_y": -44.875,
+ "pos_z": 115.65625,
+ "stations": "Daniel Barracks,Braun Station,Crown Relay"
+ },
+ {
+ "name": "Kaukai",
+ "pos_x": 35.6875,
+ "pos_y": 12.90625,
+ "pos_z": 100.875,
+ "stations": "Coulter Station,Beaumont Terminal"
+ },
+ {
+ "name": "Kaukamal",
+ "pos_x": 118.5,
+ "pos_y": -160.28125,
+ "pos_z": 59.59375,
+ "stations": "Roelofs Depot,Dingle Port,Wigura Vision"
+ },
+ {
+ "name": "Kaukamaye",
+ "pos_x": 16.1875,
+ "pos_y": -242.53125,
+ "pos_z": 90.4375,
+ "stations": "Tomita Orbital,Schwarzschild Platform"
+ },
+ {
+ "name": "Kaukamine",
+ "pos_x": 95.09375,
+ "pos_y": 4.25,
+ "pos_z": -33.71875,
+ "stations": "Vinci Station,Scully-Power Penal colony,Savinykh Hub,Blalock Enterprise"
+ },
+ {
+ "name": "Kaukas",
+ "pos_x": 97.09375,
+ "pos_y": -31.875,
+ "pos_z": -94.46875,
+ "stations": "Resnick Ring"
+ },
+ {
+ "name": "Kaukhabarra",
+ "pos_x": -8.5625,
+ "pos_y": -98.21875,
+ "pos_z": 71.5,
+ "stations": "Stone Holdings,Remec Camp"
+ },
+ {
+ "name": "Kaukhabarri",
+ "pos_x": -79.96875,
+ "pos_y": 33.71875,
+ "pos_z": -127.5,
+ "stations": "Bao's Pride"
+ },
+ {
+ "name": "Kaukhe",
+ "pos_x": 124.5625,
+ "pos_y": -57.09375,
+ "pos_z": 61.21875,
+ "stations": "Bauman Port,Younghusband Camp"
+ },
+ {
+ "name": "Kaun",
+ "pos_x": 94.6875,
+ "pos_y": -21.5,
+ "pos_z": -10.5,
+ "stations": "Artin Vision,Mills Landing,Evoluted"
+ },
+ {
+ "name": "Kaunan",
+ "pos_x": -60.96875,
+ "pos_y": 72.25,
+ "pos_z": -18.53125,
+ "stations": "Herbert Station,Levi-Civita Terminal,Hand Orbital,Ziemkiewicz Survey,Oramus Port"
+ },
+ {
+ "name": "Kaupatak",
+ "pos_x": -75.65625,
+ "pos_y": -38.9375,
+ "pos_z": -33.75,
+ "stations": "Peral Retreat"
+ },
+ {
+ "name": "Kaupolis",
+ "pos_x": 50.875,
+ "pos_y": -203.78125,
+ "pos_z": 99.8125,
+ "stations": "Shoujing Enterprise,Michael Enterprise,Mather Station,Fawcett Installation"
+ },
+ {
+ "name": "Kaupolo",
+ "pos_x": 28.90625,
+ "pos_y": -193.125,
+ "pos_z": 81.125,
+ "stations": "Smith Mines,Oberth City,Kanai Terminal,Titius Station"
+ },
+ {
+ "name": "Kaupolock",
+ "pos_x": 36.875,
+ "pos_y": -5.3125,
+ "pos_z": -43.15625,
+ "stations": "Crampton Installation,Eyharts Orbital"
+ },
+ {
+ "name": "Kaura",
+ "pos_x": 58.40625,
+ "pos_y": -13.4375,
+ "pos_z": -15.25,
+ "stations": "Howe Colony,Polansky Station,Hoshide Settlement"
+ },
+ {
+ "name": "Kaurareg",
+ "pos_x": -134.34375,
+ "pos_y": 73.625,
+ "pos_z": 46.34375,
+ "stations": "Meikle Port,Newman Lab"
+ },
+ {
+ "name": "Kaurawalaga",
+ "pos_x": 109.875,
+ "pos_y": 77.3125,
+ "pos_z": 6.78125,
+ "stations": "Palmer Depot,Gamow Orbital"
+ },
+ {
+ "name": "Kaurawish",
+ "pos_x": -77.59375,
+ "pos_y": 31.84375,
+ "pos_z": -33.34375,
+ "stations": "Kooi Hub"
+ },
+ {
+ "name": "Kaurna",
+ "pos_x": -62.4375,
+ "pos_y": -74.84375,
+ "pos_z": 111.75,
+ "stations": "Kier Settlement,Morris Platform,Stasheff Landing"
+ },
+ {
+ "name": "Kaurt",
+ "pos_x": 116.40625,
+ "pos_y": 66.59375,
+ "pos_z": 47.125,
+ "stations": "Levi-Montalcini Hub,Lee Horizons,Thagard Hub,Titov Base"
+ },
+ {
+ "name": "Kauruk",
+ "pos_x": 38.46875,
+ "pos_y": 61.34375,
+ "pos_z": 82.21875,
+ "stations": "Moore Terminal,Williams Gateway,Gaspar de Portola Dock,Baffin Co-operative,Fanning Terminal"
+ },
+ {
+ "name": "Kaurukat",
+ "pos_x": -132.46875,
+ "pos_y": -67.6875,
+ "pos_z": -24.03125,
+ "stations": "Hambly Dock,Phillifent Port,Rand Hub,Merril Penal colony"
+ },
+ {
+ "name": "Kauruku",
+ "pos_x": 85.15625,
+ "pos_y": -184.9375,
+ "pos_z": 118.1875,
+ "stations": "Axon Orbital,Mayor Colony,Selberg's Folly,Kuo Dock,Drake's Folly"
+ },
+ {
+ "name": "Kaurushi",
+ "pos_x": 47.09375,
+ "pos_y": -12.09375,
+ "pos_z": -31.40625,
+ "stations": "Alexandria Hub"
+ },
+ {
+ "name": "Kaus Australis",
+ "pos_x": 1.15625,
+ "pos_y": -25.90625,
+ "pos_z": 140.9375,
+ "stations": "Nikolayev Survey,Piaget's Claim"
+ },
+ {
+ "name": "Kaus Borealis",
+ "pos_x": -10.8125,
+ "pos_y": -9.71875,
+ "pos_z": 76.8125,
+ "stations": "Manakov Enterprise,Blalock Terminal,Libby Port"
+ },
+ {
+ "name": "Kausa",
+ "pos_x": 101.5625,
+ "pos_y": -14.40625,
+ "pos_z": -89.46875,
+ "stations": "Volta Dock,Satcher Hub,Laue Station,Russell Depot,Shelley Beacon"
+ },
+ {
+ "name": "Kausalya",
+ "pos_x": 24.625,
+ "pos_y": -39.34375,
+ "pos_z": -18.40625,
+ "stations": "Gernhardt Station,Acaba Dock,Thorne Point,Doi Ring,Agnesi City,Foda Ring,He Reach,Merchiston Town"
+ },
+ {
+ "name": "Kausan",
+ "pos_x": 25.25,
+ "pos_y": -101.6875,
+ "pos_z": 42.71875,
+ "stations": "Lyulka Ring,Panshin Vision,Williams Penal colony"
+ },
+ {
+ "name": "Kausanla",
+ "pos_x": 116.0625,
+ "pos_y": -83.90625,
+ "pos_z": 80.125,
+ "stations": "van Houten Survey,Walter Enterprise"
+ },
+ {
+ "name": "Kausasto",
+ "pos_x": -67.09375,
+ "pos_y": 110.1875,
+ "pos_z": -88.65625,
+ "stations": "Carleson Ring,Spassky Orbital,Kandel Port,Pierres Vision,Poincare Installation"
+ },
+ {
+ "name": "Kausates",
+ "pos_x": 135.34375,
+ "pos_y": -66.5625,
+ "pos_z": 85.1875,
+ "stations": "Amundsen Base,Behnisch Survey,Knight Depot,Bigourdan Orbital"
+ },
+ {
+ "name": "Kausautsu",
+ "pos_x": -120.3125,
+ "pos_y": 16.5625,
+ "pos_z": 120.59375,
+ "stations": "Taylor Dock,Herjulfsson Bastion,Tolstoi Base"
+ },
+ {
+ "name": "Kausha",
+ "pos_x": 40.78125,
+ "pos_y": -84.40625,
+ "pos_z": 41.15625,
+ "stations": "Narlikar Port,Abetti Survey,Everest Terminal,Johnson Settlement"
+ },
+ {
+ "name": "Kaushairup",
+ "pos_x": -8.4375,
+ "pos_y": 50.03125,
+ "pos_z": 58.1875,
+ "stations": "Agassiz Station,Beadle Beacon,Norman Penal colony"
+ },
+ {
+ "name": "Kaushi",
+ "pos_x": 45.09375,
+ "pos_y": 59.78125,
+ "pos_z": -102.75,
+ "stations": "Hirase Gateway,Watt Dock,Baffin Depot,Eddington Orbital,Virtanen Base"
+ },
+ {
+ "name": "Kaushpoos",
+ "pos_x": -1.90625,
+ "pos_y": 65.1875,
+ "pos_z": -79.65625,
+ "stations": "Neville Horizons,Gilliland Terminal,Verrazzano Base,Sleator Arsenal,Norgay Port"
+ },
+ {
+ "name": "Kaushu",
+ "pos_x": -42.3125,
+ "pos_y": -117.09375,
+ "pos_z": 91.125,
+ "stations": "Green Installation,Miller Terminal"
+ },
+ {
+ "name": "Kavajo",
+ "pos_x": -68.5,
+ "pos_y": 88.96875,
+ "pos_z": -109.375,
+ "stations": "Bokeili Platform"
+ },
+ {
+ "name": "Kavalan",
+ "pos_x": -144.375,
+ "pos_y": 16,
+ "pos_z": -40.25,
+ "stations": "Bakewell Settlement,Springer Landing,Kraepelin Port,Amnuel Survey"
+ },
+ {
+ "name": "Kavalliyala",
+ "pos_x": 12.65625,
+ "pos_y": -116.15625,
+ "pos_z": 122.3125,
+ "stations": "Ziewe Gateway,Unsold Orbital,Friend Depot,Sei Vision"
+ },
+ {
+ "name": "Kavane",
+ "pos_x": 72.625,
+ "pos_y": -200.90625,
+ "pos_z": 27.9375,
+ "stations": "Osterbrock Depot"
+ },
+ {
+ "name": "Kavat",
+ "pos_x": 124.875,
+ "pos_y": -61.625,
+ "pos_z": -62.96875,
+ "stations": "Chios Port,Slonczewski Dock"
+ },
+ {
+ "name": "Kawa Athi",
+ "pos_x": -47.4375,
+ "pos_y": 36.34375,
+ "pos_z": 32.15625,
+ "stations": "Bain Market,Waldeck Arsenal,Moskowitz Survey"
+ },
+ {
+ "name": "Kawal",
+ "pos_x": 56.5625,
+ "pos_y": -222.65625,
+ "pos_z": 127.28125,
+ "stations": "Parker Dock,Henderson Orbital,Litke Installation"
+ },
+ {
+ "name": "Kawarawana",
+ "pos_x": 168.25,
+ "pos_y": -124.75,
+ "pos_z": -17.78125,
+ "stations": "Reinhold Point"
+ },
+ {
+ "name": "Kawila",
+ "pos_x": 130.25,
+ "pos_y": -139.84375,
+ "pos_z": 147.53125,
+ "stations": "Lemaitre Depot,Connes Mine,Vaisala Relay"
+ },
+ {
+ "name": "Kawilocidi",
+ "pos_x": -32.53125,
+ "pos_y": -70.96875,
+ "pos_z": 77.6875,
+ "stations": "Celsius Dock,Dirac Base,Zebrowski Survey"
+ },
+ {
+ "name": "Kawilona",
+ "pos_x": -105.40625,
+ "pos_y": -94.59375,
+ "pos_z": 97,
+ "stations": "Wylie Landing"
+ },
+ {
+ "name": "Kawirun",
+ "pos_x": -119.125,
+ "pos_y": 39.5625,
+ "pos_z": -122.90625,
+ "stations": "Killough Mine,Kimberlin Landing"
+ },
+ {
+ "name": "Kaxina",
+ "pos_x": 63.65625,
+ "pos_y": -124.28125,
+ "pos_z": 118.4375,
+ "stations": "Sudworth Point,Karachkina Prospect"
+ },
+ {
+ "name": "Kaxinantu",
+ "pos_x": 63.46875,
+ "pos_y": -141.46875,
+ "pos_z": -84.5,
+ "stations": "Bingzhen Enterprise,Fraunhofer Port"
+ },
+ {
+ "name": "Kaxixo",
+ "pos_x": -36.6875,
+ "pos_y": -6.21875,
+ "pos_z": 120.96875,
+ "stations": "Nowak Hub,Kimbrough Orbital,Lawhead Vision"
+ },
+ {
+ "name": "Kayan",
+ "pos_x": -136.4375,
+ "pos_y": 34.21875,
+ "pos_z": 40.875,
+ "stations": "Herbert Retreat,Steinmuller Relay,Nansen's Progress"
+ },
+ {
+ "name": "Kayar",
+ "pos_x": -149.53125,
+ "pos_y": -28.59375,
+ "pos_z": -64.625,
+ "stations": "Puleston Colony,Fox Beacon"
+ },
+ {
+ "name": "Kayimali",
+ "pos_x": 80.25,
+ "pos_y": 4.46875,
+ "pos_z": -8.875,
+ "stations": "Schweickart Enterprise,Coppel Landing,Bouch Hub,Kelly Relay"
+ },
+ {
+ "name": "Kazahua",
+ "pos_x": 93.28125,
+ "pos_y": -180.25,
+ "pos_z": 14.6875,
+ "stations": "Rabinowitz Colony,Barmin Installation"
+ },
+ {
+ "name": "Kazatobi",
+ "pos_x": -153.28125,
+ "pos_y": -33.5,
+ "pos_z": 15.71875,
+ "stations": "Swainson Holdings"
+ },
+ {
+ "name": "Kazemlya",
+ "pos_x": -3.59375,
+ "pos_y": -166.46875,
+ "pos_z": 58.5,
+ "stations": "Lippisch Landing"
+ },
+ {
+ "name": "Kebe",
+ "pos_x": 33.375,
+ "pos_y": 9.1875,
+ "pos_z": -144.53125,
+ "stations": "Hornby Terminal"
+ },
+ {
+ "name": "Kebechs",
+ "pos_x": -120.375,
+ "pos_y": -15.90625,
+ "pos_z": 44.4375,
+ "stations": "Sharipov Base"
+ },
+ {
+ "name": "Kebechua",
+ "pos_x": 102.03125,
+ "pos_y": 106.65625,
+ "pos_z": 18.75,
+ "stations": "Pournelle Gateway,Hahn Landing,Panshin Mines,Julian Silo,Martinez Oasis"
+ },
+ {
+ "name": "Kebege",
+ "pos_x": -74.53125,
+ "pos_y": 87.59375,
+ "pos_z": -86.0625,
+ "stations": "Turtledove Dock"
+ },
+ {
+ "name": "Kebeghengi",
+ "pos_x": -72.96875,
+ "pos_y": 54.78125,
+ "pos_z": -21.15625,
+ "stations": "Jordan's Exile"
+ },
+ {
+ "name": "Kebel Yeh",
+ "pos_x": 15.71875,
+ "pos_y": -34.71875,
+ "pos_z": -99.5625,
+ "stations": "Ohm Orbital,Clayton Escape"
+ },
+ {
+ "name": "Kebeledu",
+ "pos_x": 99.15625,
+ "pos_y": -101.625,
+ "pos_z": 75.375,
+ "stations": "Bharadwaj Hub,Martiniere Terminal,Delaunay Beacon"
+ },
+ {
+ "name": "Kebes",
+ "pos_x": 83.65625,
+ "pos_y": 44.28125,
+ "pos_z": 85.84375,
+ "stations": "Kondakova Plant,Salam Base"
+ },
+ {
+ "name": "Kedo",
+ "pos_x": 27.09375,
+ "pos_y": 58,
+ "pos_z": 77.75,
+ "stations": "Mitra Installation,Yano Platform,Hannu Port"
+ },
+ {
+ "name": "Kedonen",
+ "pos_x": 112.25,
+ "pos_y": 99.625,
+ "pos_z": 67.5625,
+ "stations": "Jones Dock,McAllaster Point,Eilenberg Landing"
+ },
+ {
+ "name": "Kedones",
+ "pos_x": -43.1875,
+ "pos_y": -50.65625,
+ "pos_z": 145.84375,
+ "stations": "Bethke Colony"
+ },
+ {
+ "name": "Kedoshe",
+ "pos_x": 29.125,
+ "pos_y": 48.0625,
+ "pos_z": 79.75,
+ "stations": "Blodgett Terminal,Fettman Port,Jahn Keep,Filipchenko City,Rubruck Vision"
+ },
+ {
+ "name": "Keer",
+ "pos_x": -25.03125,
+ "pos_y": 9.71875,
+ "pos_z": -51.25,
+ "stations": "Berliner Plant"
+ },
+ {
+ "name": "Keerama",
+ "pos_x": 160.375,
+ "pos_y": -183.3125,
+ "pos_z": 40.71875,
+ "stations": "Shajn Terminal,Wilson Orbital,Joseph Delambre Port,Godwin Barracks,Bailly Vision"
+ },
+ {
+ "name": "Kehpera",
+ "pos_x": 36.96875,
+ "pos_y": -160.8125,
+ "pos_z": -3.8125,
+ "stations": "van den Hove Port,Montanari Vision,Thompson Depot,Urata Settlement,Opik Camp"
+ },
+ {
+ "name": "Kehperagwe",
+ "pos_x": -54.15625,
+ "pos_y": -72.28125,
+ "pos_z": -76.75,
+ "stations": "Acharya Depot,Hieb Port,Boming Terminal,Carson Terminal,Thagard Hub,Sharma Port,Chamitoff Station,Copernicus Port,Beltrami Point,Malyshev Station,McCaffrey Prospect,Ellern Depot,Lee Orbital"
+ },
+ {
+ "name": "Kehperi",
+ "pos_x": -30.875,
+ "pos_y": 48.65625,
+ "pos_z": 136.90625,
+ "stations": "Simmons Station,Payne Point"
+ },
+ {
+ "name": "Keiadabiko",
+ "pos_x": 31.40625,
+ "pos_y": 9.1875,
+ "pos_z": 122.09375,
+ "stations": "MacLean Vision,Bisson Gateway,MacLean Camp,Gulyaev Silo"
+ },
+ {
+ "name": "Keiadimui",
+ "pos_x": -129.875,
+ "pos_y": -57.34375,
+ "pos_z": 80.65625,
+ "stations": "Wait Colburn Orbital,Hume Gateway,Marley Bastion"
+ },
+ {
+ "name": "Keiadir",
+ "pos_x": -5.4375,
+ "pos_y": -123.71875,
+ "pos_z": 25.90625,
+ "stations": "Brorsen Landing,Fremont Arsenal"
+ },
+ {
+ "name": "Keiadiras",
+ "pos_x": 13.75,
+ "pos_y": -258.5625,
+ "pos_z": 99.4375,
+ "stations": "Humason Orbital,Kent Penal colony"
+ },
+ {
+ "name": "Keiadjara",
+ "pos_x": -114.125,
+ "pos_y": 28.90625,
+ "pos_z": -56.59375,
+ "stations": "Gagarin Installation,Payton Port,Norman Base,Mohri Vision,Shaara Horizons"
+ },
+ {
+ "name": "Keian Gu",
+ "pos_x": -16.0625,
+ "pos_y": 51.125,
+ "pos_z": -32.8125,
+ "stations": "Hahn Terminal,Nelson Orbital,Clute Prospect"
+ },
+ {
+ "name": "Keinawona",
+ "pos_x": -83.96875,
+ "pos_y": -119.21875,
+ "pos_z": 58.84375,
+ "stations": "Sharma Beacon"
+ },
+ {
+ "name": "Keinjayano",
+ "pos_x": -123.03125,
+ "pos_y": -34,
+ "pos_z": -32.09375,
+ "stations": "Haise City,Cowper Survey,Yano Survey"
+ },
+ {
+ "name": "Kekenk",
+ "pos_x": -40.5625,
+ "pos_y": -175.875,
+ "pos_z": 23.84375,
+ "stations": "Paola Dock,Lubbock Station,Kuchner Vision,al-Khowarizmi Plant"
+ },
+ {
+ "name": "Kekenks",
+ "pos_x": 22.46875,
+ "pos_y": 102.5,
+ "pos_z": 122.28125,
+ "stations": "Herrington Enterprise,Clerk Ring"
+ },
+ {
+ "name": "Kelian",
+ "pos_x": -117.375,
+ "pos_y": 5.03125,
+ "pos_z": -50.4375,
+ "stations": "Anthony de la Roche Dock"
+ },
+ {
+ "name": "Kelie",
+ "pos_x": -85.5,
+ "pos_y": -45.21875,
+ "pos_z": 85.40625,
+ "stations": "Savery Ring,Khan Market,Utley Arsenal,Elcano Relay,Werber Installation"
+ },
+ {
+ "name": "Kelin Samba",
+ "pos_x": -1.1875,
+ "pos_y": -69.71875,
+ "pos_z": 121.875,
+ "stations": "Anastase Perrotin Hub,Potocnik Hub,Kepler Station,Kidinnu Vision,Bolkow City,Huss Port,Mattei Plant,Hoyle Dock,Siegel Silo"
+ },
+ {
+ "name": "Kelish",
+ "pos_x": 95.09375,
+ "pos_y": -164.375,
+ "pos_z": 13.34375,
+ "stations": "Alphonsi Orbital,Friedrich Peters Vision,Delaunay Orbital,Tikhonravov Orbital"
+ },
+ {
+ "name": "Keliuona",
+ "pos_x": 157.6875,
+ "pos_y": -164,
+ "pos_z": 26.75,
+ "stations": "Wenzel City,Herschel Vision,Froud City,Perrine Port,Valz Terminal,Palisa Enterprise,McCrea Gateway,Krigstein Vision,Charlier Hub,Coulter Colony,Kronecker's Inheritance,Gordon Settlement,Rayhan al-Biruni Hub"
+ },
+ {
+ "name": "Keltim",
+ "pos_x": 33.84375,
+ "pos_y": -66.78125,
+ "pos_z": -42.125,
+ "stations": "Maury Dock,Laird's Progress,al-Khowarizmi Landing,Alfven Orbital,Shea City,Tomita Hub,Seitter Dock,Sitterly Orbital"
+ },
+ {
+ "name": "Kemuru",
+ "pos_x": -118.84375,
+ "pos_y": -29.3125,
+ "pos_z": -81.875,
+ "stations": "DeLucas Station,Polyakov Ring,Artsebarsky Hub,Cleve Vision,Hartlib Settlement"
+ },
+ {
+ "name": "Kemurukamar",
+ "pos_x": 70.03125,
+ "pos_y": -97.1875,
+ "pos_z": -139.34375,
+ "stations": "Grassmann Hub"
+ },
+ {
+ "name": "Kemwer",
+ "pos_x": 82.03125,
+ "pos_y": -176.125,
+ "pos_z": -3.09375,
+ "stations": "Bowen Survey,Peebles Outpost,Hopkinson Installation"
+ },
+ {
+ "name": "Kenna",
+ "pos_x": -5.5,
+ "pos_y": -100.53125,
+ "pos_z": -10.15625,
+ "stations": "Fozard Relay,Mozhaysky Gateway"
+ },
+ {
+ "name": "Kenyalivun",
+ "pos_x": -46.625,
+ "pos_y": 60.09375,
+ "pos_z": 46.15625,
+ "stations": "Ramanujan Market,Robson Keep,Bulleid Port,Bunch Vision,Noriega Survey"
+ },
+ {
+ "name": "Kenyamuna",
+ "pos_x": 24.25,
+ "pos_y": 57.6875,
+ "pos_z": -145.59375,
+ "stations": "Read Installation,Lamarck Landing,Chun Base"
+ },
+ {
+ "name": "Kepler-18",
+ "pos_x": -158.09375,
+ "pos_y": 25.25,
+ "pos_z": 30.6875,
+ "stations": "Wolfe Horizons"
+ },
+ {
+ "name": "Keraitra",
+ "pos_x": -21.625,
+ "pos_y": -48.3125,
+ "pos_z": -17.71875,
+ "stations": "Neumann Ring"
+ },
+ {
+ "name": "Kerb",
+ "pos_x": -131.75,
+ "pos_y": -91.8125,
+ "pos_z": -19.1875,
+ "stations": "Andrews Port,Lander Camp,Serling Relay,Stevens Landing"
+ },
+ {
+ "name": "Kerebaei",
+ "pos_x": 50.96875,
+ "pos_y": -67.875,
+ "pos_z": 6.9375,
+ "stations": "Eisenstein Dock,Hiroyuki Settlement,Parry Stop"
+ },
+ {
+ "name": "Keren",
+ "pos_x": 35.53125,
+ "pos_y": -103.0625,
+ "pos_z": 76.03125,
+ "stations": "Harrington Terminal,Janszoon Arsenal,Kushida Survey"
+ },
+ {
+ "name": "Kereng Dimani",
+ "pos_x": -64.46875,
+ "pos_y": -58.6875,
+ "pos_z": -141.53125,
+ "stations": "Bradshaw Relay,Nearchus Horizons"
+ },
+ {
+ "name": "Kereri",
+ "pos_x": 88.5,
+ "pos_y": -103,
+ "pos_z": 139.5625,
+ "stations": "Baynes Station,Giger Orbital,Bessel Terminal,Yourkevitch Enterprise,Lasswitz Survey"
+ },
+ {
+ "name": "Keries",
+ "pos_x": -18.90625,
+ "pos_y": 27.21875,
+ "pos_z": 12.59375,
+ "stations": "Derrickson's Escape,Almagro Settlement"
+ },
+ {
+ "name": "Ketekhmet",
+ "pos_x": 9.90625,
+ "pos_y": -102.5,
+ "pos_z": -23.4375,
+ "stations": "Evans Base,Thurston Refinery,Zubrin Installation,Archer Refinery"
+ },
+ {
+ "name": "Ketsauts",
+ "pos_x": 37.25,
+ "pos_y": -139.125,
+ "pos_z": -12.46875,
+ "stations": "Proctor Plant,Wilhelm von Struve Beacon,Armero Landing,Eudoxus Beacon"
+ },
+ {
+ "name": "Ketse Aibe",
+ "pos_x": 100.3125,
+ "pos_y": -162.28125,
+ "pos_z": 137,
+ "stations": "Kerimov Outpost,Kemurdzhian Barracks"
+ },
+ {
+ "name": "Ketsegezi",
+ "pos_x": 61.59375,
+ "pos_y": -3.46875,
+ "pos_z": -102.8125,
+ "stations": "Chiao Settlement,Bosch Beacon,Kazantsev Base"
+ },
+ {
+ "name": "Khaima",
+ "pos_x": -23.625,
+ "pos_y": -10.75,
+ "pos_z": -89.46875,
+ "stations": "Kondakova City,Artyukhin Terminal,Hackworth Enterprise"
+ },
+ {
+ "name": "Khaithu",
+ "pos_x": 72.03125,
+ "pos_y": -70.1875,
+ "pos_z": 110.78125,
+ "stations": "Seyfert Platform,Backlund Terminal"
+ },
+ {
+ "name": "Khaka",
+ "pos_x": 40.21875,
+ "pos_y": -9.78125,
+ "pos_z": 38.46875,
+ "stations": "Qureshi Enterprise,Love Landing,Ford Hub,Hill Arsenal,Hutton Dock"
+ },
+ {
+ "name": "Khakareng",
+ "pos_x": 45.09375,
+ "pos_y": -101.15625,
+ "pos_z": -97.15625,
+ "stations": "Rabinowitz Settlement,Biesbroeck Hub,Nansen Landing"
+ },
+ {
+ "name": "Khakari",
+ "pos_x": 113,
+ "pos_y": -16.71875,
+ "pos_z": -73.625,
+ "stations": "Murdoch Dock,Makarov Base"
+ },
+ {
+ "name": "Khakha",
+ "pos_x": 23.6875,
+ "pos_y": 101.65625,
+ "pos_z": 58.6875,
+ "stations": "Coelho Settlement,Strekalov Settlement,Ramsay Dock"
+ },
+ {
+ "name": "Khakmo",
+ "pos_x": 78.59375,
+ "pos_y": -4.53125,
+ "pos_z": 47.59375,
+ "stations": "Moore City,Butcher Relay,Gromov Barracks"
+ },
+ {
+ "name": "Khakum",
+ "pos_x": 88.09375,
+ "pos_y": -176.90625,
+ "pos_z": 59.34375,
+ "stations": "Danforth Mines"
+ },
+ {
+ "name": "Khakya",
+ "pos_x": 192.875,
+ "pos_y": -140.15625,
+ "pos_z": 96.53125,
+ "stations": "Fox Colony,Plaskett Landing,Kornbluth Holdings,Puiseux's Progress"
+ },
+ {
+ "name": "Khaman",
+ "pos_x": -48.21875,
+ "pos_y": -122,
+ "pos_z": -13.34375,
+ "stations": "Muramatsu Dock,McDevitt Enterprise,Soper Silo"
+ },
+ {
+ "name": "Khamataman",
+ "pos_x": 55.21875,
+ "pos_y": -242.65625,
+ "pos_z": -30.3125,
+ "stations": "Wisdom Vision,Drake Orbital,Safdie Terminal,Corte-Real Plant,Cassini Vision"
+ },
+ {
+ "name": "Khambara",
+ "pos_x": 21.59375,
+ "pos_y": 56.34375,
+ "pos_z": -164.9375,
+ "stations": "Macgregor Station,Ocampo Silo"
+ },
+ {
+ "name": "Khamoori",
+ "pos_x": -50,
+ "pos_y": 86.84375,
+ "pos_z": -131.25,
+ "stations": "Shiner Hub,White Vision,Maybury Landing"
+ },
+ {
+ "name": "Khampti",
+ "pos_x": 50.1875,
+ "pos_y": -147.96875,
+ "pos_z": 46.5625,
+ "stations": "Babcock Gateway,Milne Gateway,Kushida Vision,Wafa Hub,Fabian Dock,Olahus Hub,Lozino-Lozinskiy Ring,Wagner Palace,Bailey Observatory,Izumikawa City,Phillips Terminal,Peirce Refinery,McIntosh's Claim"
+ },
+ {
+ "name": "Khan",
+ "pos_x": 4.75,
+ "pos_y": -49.4375,
+ "pos_z": 94.15625,
+ "stations": "Bell Market,Robinson Hub,Burnet Ring,Mackenzie Point,Behring Hub,Khan Vision,Buffett City,Nelder Vision,Sturgeon Base"
+ },
+ {
+ "name": "Khan Gubii",
+ "pos_x": 95.4375,
+ "pos_y": -12.90625,
+ "pos_z": 125.8125,
+ "stations": "Alpers City,Noli Point"
+ },
+ {
+ "name": "Khanci",
+ "pos_x": -5.5,
+ "pos_y": 99.3125,
+ "pos_z": 82.03125,
+ "stations": "Bloomfield Colony,Tani Colony,Fawcett Survey"
+ },
+ {
+ "name": "Khants",
+ "pos_x": -125.21875,
+ "pos_y": 52.375,
+ "pos_z": 55.8125,
+ "stations": "Julian Terminal,Hartsfield Plant"
+ },
+ {
+ "name": "Kharahua",
+ "pos_x": 164.03125,
+ "pos_y": 31.9375,
+ "pos_z": 14.9375,
+ "stations": "Lorentz Station"
+ },
+ {
+ "name": "Kharaitis",
+ "pos_x": 127.65625,
+ "pos_y": -114.625,
+ "pos_z": 15.875,
+ "stations": "Barbuy Orbital,Stein Terminal,Hampson Vision"
+ },
+ {
+ "name": "Kharaka",
+ "pos_x": -1.75,
+ "pos_y": -219.1875,
+ "pos_z": 10.90625,
+ "stations": "Impey Settlement,Dassault Beacon,Egan Mine"
+ },
+ {
+ "name": "Kharakas",
+ "pos_x": 22.40625,
+ "pos_y": -225.625,
+ "pos_z": 28.21875,
+ "stations": "Schommer Terminal,Binney's Progress,Encke Depot"
+ },
+ {
+ "name": "Khari",
+ "pos_x": 120.78125,
+ "pos_y": -73.34375,
+ "pos_z": 20.875,
+ "stations": "Huggins Hub,Salpeter Orbital,van de Sande Bakhuyzen Depot"
+ },
+ {
+ "name": "Kharinda",
+ "pos_x": -32.46875,
+ "pos_y": 42.625,
+ "pos_z": 39.125,
+ "stations": "Rontgen's Progress,Chadwick Orbital,Chargaff Hub"
+ },
+ {
+ "name": "Kharpulo",
+ "pos_x": 41.9375,
+ "pos_y": -155.6875,
+ "pos_z": 16.3125,
+ "stations": "Somerville Station,Blaylock Enterprise,Alexandria City,Simmons Silo"
+ },
+ {
+ "name": "Kharu",
+ "pos_x": 128.15625,
+ "pos_y": -51.875,
+ "pos_z": -133.34375,
+ "stations": "Doctorow Beacon,Flinders Terminal,Harrison Silo"
+ },
+ {
+ "name": "Kharvii",
+ "pos_x": -55.84375,
+ "pos_y": -161,
+ "pos_z": -11.65625,
+ "stations": "Birmingham Dock,Pippin Dock,Elwood Dock,Forsskal Hub"
+ },
+ {
+ "name": "Kharviri",
+ "pos_x": 50.8125,
+ "pos_y": 71.71875,
+ "pos_z": 130.8125,
+ "stations": "Andrews' Exile,Dukaj Settlement"
+ },
+ {
+ "name": "Kharwa",
+ "pos_x": -55.59375,
+ "pos_y": -98.53125,
+ "pos_z": -97.25,
+ "stations": "Glashow Station"
+ },
+ {
+ "name": "Kharwar",
+ "pos_x": 38.9375,
+ "pos_y": -180.8125,
+ "pos_z": -83.59375,
+ "stations": "Stechkin Depot"
+ },
+ {
+ "name": "Khary",
+ "pos_x": 93.96875,
+ "pos_y": -241.78125,
+ "pos_z": 93.96875,
+ "stations": "Arnold Survey,Giger Enterprise,Herschel Orbital,McIntyre Vision,Rothman Vista"
+ },
+ {
+ "name": "Kharyi",
+ "pos_x": -58.75,
+ "pos_y": -136.875,
+ "pos_z": 11.53125,
+ "stations": "Duckworth Port,Georg Bothe Enterprise"
+ },
+ {
+ "name": "Khasa",
+ "pos_x": -97.09375,
+ "pos_y": 75.65625,
+ "pos_z": 8.71875,
+ "stations": "Tull Terminal,Levi-Civita Enterprise"
+ },
+ {
+ "name": "Khasargiz",
+ "pos_x": 128.125,
+ "pos_y": -81.4375,
+ "pos_z": 130.0625,
+ "stations": "Phillips Vision,Hand's Inheritance,Jean Dock,Jordan's Inheritance"
+ },
+ {
+ "name": "Khasia",
+ "pos_x": -31.5625,
+ "pos_y": -62.03125,
+ "pos_z": -136.90625,
+ "stations": "Chern Dock,Hill Prospect"
+ },
+ {
+ "name": "Khasiri",
+ "pos_x": 76.59375,
+ "pos_y": 76.59375,
+ "pos_z": -40.0625,
+ "stations": "Matteucci Terminal,Kuipers Port,Gutierrez Port,Stevens Exchange"
+ },
+ {
+ "name": "Khawathlang",
+ "pos_x": 27.34375,
+ "pos_y": -2.125,
+ "pos_z": 114.71875,
+ "stations": "Bouch Settlement,Ivanishin Laboratory,Tesla Landing"
+ },
+ {
+ "name": "Khawchuang",
+ "pos_x": -45.8125,
+ "pos_y": -15.59375,
+ "pos_z": 81.15625,
+ "stations": "Hartsfield Landing,Willis Terminal,Freycinet Depot"
+ },
+ {
+ "name": "Khelma",
+ "pos_x": -78.125,
+ "pos_y": -3.21875,
+ "pos_z": 26.84375,
+ "stations": "Kidman Hub,Lucid Orbital,Jones Settlement"
+ },
+ {
+ "name": "Khemaraui",
+ "pos_x": 203.0625,
+ "pos_y": -175.28125,
+ "pos_z": 85.5625,
+ "stations": "Schomburg Base,Metcalf Point,Covington's Folly"
+ },
+ {
+ "name": "Khencani",
+ "pos_x": 90.34375,
+ "pos_y": -174.90625,
+ "pos_z": 121.53125,
+ "stations": "Fairey Stop,Lowry Arsenal"
+ },
+ {
+ "name": "Khengu",
+ "pos_x": -49,
+ "pos_y": -1.84375,
+ "pos_z": 132.8125,
+ "stations": "Fullerton Platform"
+ },
+ {
+ "name": "Khep No Zi",
+ "pos_x": 142.9375,
+ "pos_y": -93,
+ "pos_z": 122.15625,
+ "stations": "Schulhof Prospect,Russell Terminal,Ritchey Landing,Beltrami Enterprise,Gardner Arsenal"
+ },
+ {
+ "name": "Khepeche",
+ "pos_x": 49.6875,
+ "pos_y": 47.1875,
+ "pos_z": 146,
+ "stations": "Nemere Enterprise,Gresley Survey,Freud Colony"
+ },
+ {
+ "name": "Khepehueno",
+ "pos_x": 115.28125,
+ "pos_y": -67.6875,
+ "pos_z": 64.4375,
+ "stations": "Kranz Dock"
+ },
+ {
+ "name": "Khepera",
+ "pos_x": 64.09375,
+ "pos_y": -71.75,
+ "pos_z": 86.5625,
+ "stations": "Very Horizons,Hogg Depot"
+ },
+ {
+ "name": "Khepertha",
+ "pos_x": -17.8125,
+ "pos_y": 20.75,
+ "pos_z": 114.96875,
+ "stations": "Lavoisier Survey,Kepler Vision,Roberts Refinery"
+ },
+ {
+ "name": "Khepri",
+ "pos_x": 36.0625,
+ "pos_y": 5.5625,
+ "pos_z": 39.0625,
+ "stations": "Banks Port,Gutierrez City,Newton Port,Hooke City,Guidoni Orbital,Oxley Base,Kettle Base,Bernard Orbital"
+ },
+ {
+ "name": "Kher",
+ "pos_x": -11.5,
+ "pos_y": 42.4375,
+ "pos_z": -104.40625,
+ "stations": "Arkwright Port,Creamer Ring,Godwin Station"
+ },
+ {
+ "name": "Khered",
+ "pos_x": -52.03125,
+ "pos_y": -71.625,
+ "pos_z": -35.78125,
+ "stations": "Davy Gateway,Townshend Gateway,Farouk Survey,Lyakhov Penal colony,Sanger Station,Manakov City,Lebedev Ring,Rocklynne Refinery"
+ },
+ {
+ "name": "Kheret",
+ "pos_x": 60.65625,
+ "pos_y": -173.1875,
+ "pos_z": -76.5625,
+ "stations": "Denning Terminal,Otus Survey,Akiyama Landing"
+ },
+ {
+ "name": "Kheretii",
+ "pos_x": -0.75,
+ "pos_y": -59.4375,
+ "pos_z": 132.96875,
+ "stations": "Chebyshev Port,Wisniewski-Snerg Hub,Wilson Horizons,Weber Orbital,Hambly Horizons,Watts Terminal,Hoffman Port"
+ },
+ {
+ "name": "Khernidjal",
+ "pos_x": -35.9375,
+ "pos_y": 70.5625,
+ "pos_z": -7.65625,
+ "stations": "West Gateway,Brooks Enterprise,Morris Dock,Moresby Terminal,McMullen Beacon"
+ },
+ {
+ "name": "Kherno",
+ "pos_x": 128.875,
+ "pos_y": -17.59375,
+ "pos_z": -60.9375,
+ "stations": "Flint Settlement"
+ },
+ {
+ "name": "Khertamoana",
+ "pos_x": -112.46875,
+ "pos_y": 33.125,
+ "pos_z": 8.625,
+ "stations": "J. G. Ballard Hub,Ricci Point"
+ },
+ {
+ "name": "Kherthaje",
+ "pos_x": 105.625,
+ "pos_y": 12.78125,
+ "pos_z": 72.78125,
+ "stations": "Samuda Point,Herreshoff Prospect"
+ },
+ {
+ "name": "Khitibe",
+ "pos_x": -19.75,
+ "pos_y": -106.65625,
+ "pos_z": 103.875,
+ "stations": "Cabot Depot,Killough Base"
+ },
+ {
+ "name": "Khnemurt",
+ "pos_x": -8.5625,
+ "pos_y": -71.34375,
+ "pos_z": 54,
+ "stations": "Shull Settlement,Bosch Point"
+ },
+ {
+ "name": "Khnum",
+ "pos_x": 49.8125,
+ "pos_y": -14.84375,
+ "pos_z": -14.0625,
+ "stations": "Bowersox Ring,Horowitz Installation,Dana City,Akiyama Station,Pogue's Claim,Sturckow Ring,Kondratyev Terminal,Wylie Beacon"
+ },
+ {
+ "name": "Khodhis",
+ "pos_x": -19.28125,
+ "pos_y": -33.9375,
+ "pos_z": -68.375,
+ "stations": "Perez Dock,Smith Survey,Wafer Lab"
+ },
+ {
+ "name": "Khodia",
+ "pos_x": -98.8125,
+ "pos_y": -99.6875,
+ "pos_z": 65.8125,
+ "stations": "Kessel's Inheritance,Brule Vision,Pordenone Survey"
+ },
+ {
+ "name": "Khodiwobogi",
+ "pos_x": 21.96875,
+ "pos_y": -64.46875,
+ "pos_z": 60.9375,
+ "stations": "Chadwick Terminal,Sunyaev Installation"
+ },
+ {
+ "name": "Khodur",
+ "pos_x": 62.8125,
+ "pos_y": -244.03125,
+ "pos_z": 65.09375,
+ "stations": "Poyser Mine"
+ },
+ {
+ "name": "Kholedo",
+ "pos_x": -95.09375,
+ "pos_y": 33.5625,
+ "pos_z": -7.15625,
+ "stations": "Asaro Dock,Tapinas Barracks,Lopez de Villalobos Colony"
+ },
+ {
+ "name": "Kholevi",
+ "pos_x": -1.5625,
+ "pos_y": -55.40625,
+ "pos_z": 117.65625,
+ "stations": "Al Sufi Enterprise,Turzillo's Progress,Beaufoy Installation"
+ },
+ {
+ "name": "Kholhou",
+ "pos_x": 14.09375,
+ "pos_y": -177.875,
+ "pos_z": 1.9375,
+ "stations": "McCool Station,Payne-Gaposchkin Port,Lubbock Ring,Kuchemann Vision,Furukawa Ring"
+ },
+ {
+ "name": "Kholhoujem",
+ "pos_x": 81.96875,
+ "pos_y": 55.34375,
+ "pos_z": -117.5625,
+ "stations": "Einstein Survey,Titov Horizons"
+ },
+ {
+ "name": "Kholhouvun",
+ "pos_x": 15.65625,
+ "pos_y": 84.71875,
+ "pos_z": 120.90625,
+ "stations": "Thorne Landing,Ryumin Colony"
+ },
+ {
+ "name": "Kholita",
+ "pos_x": -155.03125,
+ "pos_y": 23.46875,
+ "pos_z": 81.34375,
+ "stations": "Dampier Enterprise,Whitelaw Port,Liouville Orbital,Lewitt Hub,Rochon Reach"
+ },
+ {
+ "name": "Kholo",
+ "pos_x": 4.96875,
+ "pos_y": -8.28125,
+ "pos_z": 98.21875,
+ "stations": "Tucker City,Jiushao Laboratory"
+ },
+ {
+ "name": "Kholu",
+ "pos_x": 15.15625,
+ "pos_y": -139.46875,
+ "pos_z": -23.9375,
+ "stations": "Abetti Vision,Bauschinger Relay,Barbuy Landing,Brunner Ring,Kosai Station"
+ },
+ {
+ "name": "Kholul",
+ "pos_x": -46.9375,
+ "pos_y": -7.84375,
+ "pos_z": -152.1875,
+ "stations": "Mitchell Landing,Silverberg Orbital,Lynn Prospect,Eckford Survey"
+ },
+ {
+ "name": "Khona",
+ "pos_x": -123.46875,
+ "pos_y": 119.21875,
+ "pos_z": -58.46875,
+ "stations": "Ziemianski Enterprise,Kube-McDowell Orbital,McAuley Keep,Fuchs Prospect"
+ },
+ {
+ "name": "Khond",
+ "pos_x": -31.84375,
+ "pos_y": 121.625,
+ "pos_z": 61.84375,
+ "stations": "Vancouver Beacon,Forbes Relay,Ogden Depot"
+ },
+ {
+ "name": "Khondalini",
+ "pos_x": 117.25,
+ "pos_y": -200.34375,
+ "pos_z": 17.21875,
+ "stations": "Wachmann Terminal"
+ },
+ {
+ "name": "Khondo Po",
+ "pos_x": -119.71875,
+ "pos_y": 39.46875,
+ "pos_z": -93.25,
+ "stations": "Dezhurov Terminal,Beregovoi Settlement,Oersted Depot"
+ },
+ {
+ "name": "Khones",
+ "pos_x": -42.84375,
+ "pos_y": -18.15625,
+ "pos_z": 137,
+ "stations": "Henricks Colony,Velazquez Dock"
+ },
+ {
+ "name": "Khonesti",
+ "pos_x": 75.6875,
+ "pos_y": -204.875,
+ "pos_z": 40.5,
+ "stations": "West Port,Hermite Penal colony,Koolhaas Station"
+ },
+ {
+ "name": "Khong Shi",
+ "pos_x": -104.15625,
+ "pos_y": -15.53125,
+ "pos_z": 105.25,
+ "stations": "Blish Prospect"
+ },
+ {
+ "name": "Khong Wang",
+ "pos_x": 66.21875,
+ "pos_y": -11.28125,
+ "pos_z": -44.5,
+ "stations": "Stapledon Dock,Maler's Folly"
+ },
+ {
+ "name": "Khongalis",
+ "pos_x": -95.625,
+ "pos_y": 8.125,
+ "pos_z": -36.3125,
+ "stations": "Taylor Co-operative"
+ },
+ {
+ "name": "Khongarun",
+ "pos_x": 172.9375,
+ "pos_y": -136.65625,
+ "pos_z": 102,
+ "stations": "Mrkos Colony,Bahcall Hub"
+ },
+ {
+ "name": "Khongyan",
+ "pos_x": 110.75,
+ "pos_y": -64.71875,
+ "pos_z": 110,
+ "stations": "Louis de Lacaille Platform,Mueller Plant"
+ },
+ {
+ "name": "Khonsa",
+ "pos_x": 20,
+ "pos_y": -116.8125,
+ "pos_z": -35.375,
+ "stations": "Lalande Station,Rankin Arsenal,Coggia Mines"
+ },
+ {
+ "name": "Khonse",
+ "pos_x": -28.71875,
+ "pos_y": -136.59375,
+ "pos_z": 97.125,
+ "stations": "Lilienthal Terminal,Apianus' Inheritance"
+ },
+ {
+ "name": "Khontam",
+ "pos_x": 160.0625,
+ "pos_y": 42.9375,
+ "pos_z": -11.3125,
+ "stations": "Judson Dock,Kubasov Beacon"
+ },
+ {
+ "name": "Khonti",
+ "pos_x": 150.84375,
+ "pos_y": -206.84375,
+ "pos_z": -12.46875,
+ "stations": "Cummings Vision,Amnuel Enterprise,South Station,Kibalchich Station"
+ },
+ {
+ "name": "Khowa",
+ "pos_x": -49.25,
+ "pos_y": 51.3125,
+ "pos_z": -140.9375,
+ "stations": "Ostwald Platform,Bayliss Penal colony"
+ },
+ {
+ "name": "Khowahing",
+ "pos_x": -130.875,
+ "pos_y": -77.6875,
+ "pos_z": -87.625,
+ "stations": "Buckey Terminal,Jenner's Inheritance,Chu Enterprise,Bella Terminal,Toll Hub"
+ },
+ {
+ "name": "Khowatsu",
+ "pos_x": 54.03125,
+ "pos_y": 87.90625,
+ "pos_z": 12.09375,
+ "stations": "Haldeman Installation,Smith Port"
+ },
+ {
+ "name": "Khowei",
+ "pos_x": 149.71875,
+ "pos_y": -127.71875,
+ "pos_z": -73.53125,
+ "stations": "De Horizons,Howe Port,Palitzsch Terminal,Smith Arsenal"
+ },
+ {
+ "name": "Khronos",
+ "pos_x": 4.03125,
+ "pos_y": -4.5625,
+ "pos_z": 57.90625,
+ "stations": "Evans Hub,Walter Refinery"
+ },
+ {
+ "name": "Khrudiyu",
+ "pos_x": -10.28125,
+ "pos_y": -2.3125,
+ "pos_z": 164.125,
+ "stations": "Conway Landing,Cartier Enterprise"
+ },
+ {
+ "name": "Khruttones",
+ "pos_x": -14.59375,
+ "pos_y": -142.3125,
+ "pos_z": -105.78125,
+ "stations": "Morgan Port,Hahn Orbital,Metcalf Dock,Reed Plant,Lanier Landing"
+ },
+ {
+ "name": "Khruva",
+ "pos_x": -16.875,
+ "pos_y": 128.625,
+ "pos_z": 60.65625,
+ "stations": "Ramelli Terminal,Xing Settlement"
+ },
+ {
+ "name": "Khruvanaye",
+ "pos_x": -0.375,
+ "pos_y": 40.5,
+ "pos_z": 119.9375,
+ "stations": "Burbank Mine"
+ },
+ {
+ "name": "Khruvandji",
+ "pos_x": 5.09375,
+ "pos_y": -120.8125,
+ "pos_z": 12.28125,
+ "stations": "Consolmagno Enterprise,Abel Depot,Julian's Progress"
+ },
+ {
+ "name": "Khruvani",
+ "pos_x": -40.46875,
+ "pos_y": 8.3125,
+ "pos_z": -126.0625,
+ "stations": "Disch Station,Pike City,Slonczewski Depot,Carlisle Gateway"
+ },
+ {
+ "name": "Khun",
+ "pos_x": -171.59375,
+ "pos_y": 19.96875,
+ "pos_z": -56.96875,
+ "stations": "Long Sight Base,Alexandrov Dock,Gunn Laboratory,Cremona Prospect"
+ },
+ {
+ "name": "Khuzwan Zu",
+ "pos_x": 46.625,
+ "pos_y": -117.96875,
+ "pos_z": -115.25,
+ "stations": "Macdonald Settlement"
+ },
+ {
+ "name": "Khuzwane",
+ "pos_x": 31.96875,
+ "pos_y": -58.9375,
+ "pos_z": -103.25,
+ "stations": "O'Donnell Station,Brackett Camp,Fiennes Port,Mallory Arsenal"
+ },
+ {
+ "name": "Khuzwangpo",
+ "pos_x": -84.03125,
+ "pos_y": 16.125,
+ "pos_z": -97.21875,
+ "stations": "Bursch Landing,Fontana Dock"
+ },
+ {
+ "name": "Khwal",
+ "pos_x": 137.5,
+ "pos_y": 17.75,
+ "pos_z": -95.84375,
+ "stations": "Metz Settlement,Piccard Terminal,Stein Depot"
+ },
+ {
+ "name": "Khwar",
+ "pos_x": -58.46875,
+ "pos_y": -11.9375,
+ "pos_z": -116.53125,
+ "stations": "Roberts Dock,Bierce Beacon,Kingsmill Plant"
+ },
+ {
+ "name": "Khwarakan",
+ "pos_x": 43.28125,
+ "pos_y": -237.84375,
+ "pos_z": 21.53125,
+ "stations": "Chertok Hub,Thollon Platform,Gwynn Installation"
+ },
+ {
+ "name": "Khwathis",
+ "pos_x": 90.625,
+ "pos_y": 82.5625,
+ "pos_z": -109.5625,
+ "stations": "Morrison Enterprise,Levinson Station,Julian Port,Moran Prospect,Selous Prospect"
+ },
+ {
+ "name": "Khwatka",
+ "pos_x": -109.875,
+ "pos_y": 76.21875,
+ "pos_z": -16.25,
+ "stations": "Hernandez Dock,Linge Installation,Hambly Base,Rushd Gateway"
+ },
+ {
+ "name": "Ki",
+ "pos_x": -16.78125,
+ "pos_y": -29.15625,
+ "pos_z": -65.15625,
+ "stations": "Binet Port,Horowitz Orbital,Isherwood Installation,Burkin Horizons,McBride Vision,Hammond Vision,Gibson Hub,Ashby Port,Weber Terminal,Simpson City,Mohmand Hub,Pawelczyk Enterprise"
+ },
+ {
+ "name": "Ki Lao Kana",
+ "pos_x": 147.125,
+ "pos_y": -117.8125,
+ "pos_z": 86.625,
+ "stations": "Dixon Landing,Shute Station,Busch Landing"
+ },
+ {
+ "name": "Ki Laufey",
+ "pos_x": 164.5,
+ "pos_y": -14.25,
+ "pos_z": -5.34375,
+ "stations": "Harness Landing,Fiennes Orbital,Napier Mines,Khayyam Colony"
+ },
+ {
+ "name": "Kians",
+ "pos_x": 28.53125,
+ "pos_y": 27.375,
+ "pos_z": 120.6875,
+ "stations": "Vizcaino Dock"
+ },
+ {
+ "name": "Kicho",
+ "pos_x": -97.53125,
+ "pos_y": -32.09375,
+ "pos_z": 52.96875,
+ "stations": "Lowry Landing,Drebbel Landing"
+ },
+ {
+ "name": "Kidi",
+ "pos_x": -132.3125,
+ "pos_y": -16.53125,
+ "pos_z": -93.1875,
+ "stations": "Oliver Settlement,Shonin Holdings"
+ },
+ {
+ "name": "Kidil",
+ "pos_x": -12,
+ "pos_y": -152.0625,
+ "pos_z": 60.28125,
+ "stations": "Hahn Survey,Kopff Holdings,Maury Orbital"
+ },
+ {
+ "name": "Kidiuhet",
+ "pos_x": 64.71875,
+ "pos_y": 148.59375,
+ "pos_z": 4.71875,
+ "stations": "Doi Landing,Boas Dock,Gillekens Colony"
+ },
+ {
+ "name": "Kiga",
+ "pos_x": 45.96875,
+ "pos_y": 41.53125,
+ "pos_z": -82.375,
+ "stations": "Gernhardt Hub,Onnes Dock,Burke Survey,Tilley Penal colony"
+ },
+ {
+ "name": "Kigaedu",
+ "pos_x": -40.15625,
+ "pos_y": -32.625,
+ "pos_z": 172.4375,
+ "stations": "Schmidt Landing,Ray Arena"
+ },
+ {
+ "name": "Kigagua",
+ "pos_x": -55.46875,
+ "pos_y": 5.28125,
+ "pos_z": 5,
+ "stations": "Drexler Colony,Levchenko Mines,Beekman's Inheritance"
+ },
+ {
+ "name": "Kigah",
+ "pos_x": 120.15625,
+ "pos_y": 43.6875,
+ "pos_z": 93.625,
+ "stations": "Key Legacy"
+ },
+ {
+ "name": "Kigalky",
+ "pos_x": 137.8125,
+ "pos_y": -60.4375,
+ "pos_z": -81.15625,
+ "stations": "Ferguson Asylum"
+ },
+ {
+ "name": "Kigana",
+ "pos_x": -13.09375,
+ "pos_y": -8.625,
+ "pos_z": 118.5625,
+ "stations": "Bennett Orbital,Grechko City,Thornton Colony,Chasles Point"
+ },
+ {
+ "name": "Kigandan",
+ "pos_x": 7.5625,
+ "pos_y": -72.25,
+ "pos_z": 10.75,
+ "stations": "Vinogradov Port,Bulleid Ring,Bethe Dock"
+ },
+ {
+ "name": "Kigaso",
+ "pos_x": -118.96875,
+ "pos_y": 76.53125,
+ "pos_z": -141.5,
+ "stations": "Barentsz Base"
+ },
+ {
+ "name": "Kikab",
+ "pos_x": 163.5,
+ "pos_y": -181.5,
+ "pos_z": 87.40625,
+ "stations": "Wirtanen Point"
+ },
+ {
+ "name": "Kikapu",
+ "pos_x": -29,
+ "pos_y": -63.375,
+ "pos_z": 137.84375,
+ "stations": "Barreiros Ring,Palmer Prospect"
+ },
+ {
+ "name": "Kikas",
+ "pos_x": -113.40625,
+ "pos_y": -5.3125,
+ "pos_z": 126.84375,
+ "stations": "Crown Terminal,Fisher Orbital,Al-Jazari Plant"
+ },
+ {
+ "name": "Kikians",
+ "pos_x": 69.4375,
+ "pos_y": -191.90625,
+ "pos_z": 101.8125,
+ "stations": "Seki Station,Cottenot Terminal,Legendre Hub"
+ },
+ {
+ "name": "Kikua",
+ "pos_x": 61.25,
+ "pos_y": -76.96875,
+ "pos_z": 27.03125,
+ "stations": "Finlay-Freundlich Hub,Stevenson Orbital,Gibson Survey,Lopez-Garcia Camp,Elder Camp"
+ },
+ {
+ "name": "Kilinigua",
+ "pos_x": 37.0625,
+ "pos_y": 1.4375,
+ "pos_z": 139.59375,
+ "stations": "Chargaff Arsenal,Napier Dock,Creighton Works"
+ },
+ {
+ "name": "Kilique",
+ "pos_x": 90.1875,
+ "pos_y": -264.375,
+ "pos_z": 7,
+ "stations": "Hirayama Point"
+ },
+ {
+ "name": "Kiliwatsuki",
+ "pos_x": 24.625,
+ "pos_y": 34.9375,
+ "pos_z": 100.90625,
+ "stations": "Walz Gateway"
+ },
+ {
+ "name": "Killke",
+ "pos_x": -127.28125,
+ "pos_y": -21.71875,
+ "pos_z": -56.9375,
+ "stations": "Clifford Horizons,Tavares Outpost,King Base"
+ },
+ {
+ "name": "Kim 2-17",
+ "pos_x": -12.28125,
+ "pos_y": -30.15625,
+ "pos_z": -111.21875,
+ "stations": "Thomas Vision,White Settlement,Rutherford Landing"
+ },
+ {
+ "name": "Kimi",
+ "pos_x": -84.84375,
+ "pos_y": 91.15625,
+ "pos_z": -20.78125,
+ "stations": "Mackenzie Station,Gregory Reach,Brooks Barracks,Regiomontanus Observatory"
+ },
+ {
+ "name": "Kimici",
+ "pos_x": -154.8125,
+ "pos_y": -64.71875,
+ "pos_z": 25.84375,
+ "stations": "Roberts Terminal,Dowling Prospect,Petaja Settlement"
+ },
+ {
+ "name": "Kimih",
+ "pos_x": 108.8125,
+ "pos_y": -113.21875,
+ "pos_z": 126.78125,
+ "stations": "Lowell Station"
+ },
+ {
+ "name": "Kimir",
+ "pos_x": -44.9375,
+ "pos_y": 49.5,
+ "pos_z": -130.8125,
+ "stations": "Savery Outpost"
+ },
+ {
+ "name": "Kin",
+ "pos_x": -99.90625,
+ "pos_y": 35.75,
+ "pos_z": 75.8125,
+ "stations": "Kotzebue Landing,Vries' Folly,Shaikh Mines,Grigson Escape"
+ },
+ {
+ "name": "Kina",
+ "pos_x": 137.21875,
+ "pos_y": 18.125,
+ "pos_z": 87.375,
+ "stations": "Hedin Plant,Fox Arena,Gwynn Base"
+ },
+ {
+ "name": "Kinago",
+ "pos_x": -67.40625,
+ "pos_y": -7.40625,
+ "pos_z": 150.0625,
+ "stations": "Silva Settlement,Fozard Ring,Caidin Hub,Sinisalo Hub"
+ },
+ {
+ "name": "Kinesi",
+ "pos_x": -9510.3125,
+ "pos_y": -895.53125,
+ "pos_z": 19799.875,
+ "stations": "Crevie's Salvo,Mortimer's Charm,Dallin's Boondocks"
+ },
+ {
+ "name": "Kini",
+ "pos_x": -47.125,
+ "pos_y": -3.25,
+ "pos_z": 60.28125,
+ "stations": "Kadenyuk Orbital"
+ },
+ {
+ "name": "Kinna",
+ "pos_x": 29.28125,
+ "pos_y": -89.5625,
+ "pos_z": 115.8125,
+ "stations": "Pogson Station,Chertovsky Prospect"
+ },
+ {
+ "name": "Kinnara",
+ "pos_x": 100.96875,
+ "pos_y": 71.40625,
+ "pos_z": -35.0625,
+ "stations": "Grechko Refinery,Ayerdhal Horizons"
+ },
+ {
+ "name": "Kinne",
+ "pos_x": 48.25,
+ "pos_y": -105.28125,
+ "pos_z": -28.71875,
+ "stations": "Linge Refinery,Apianus Station,Dreyer Holdings"
+ },
+ {
+ "name": "Kioskurber",
+ "pos_x": -52.84375,
+ "pos_y": 27.71875,
+ "pos_z": -73,
+ "stations": "Mohmand Orbital,Wiberg Station,Voss Beacon,Andreas Ring,Frick Penal colony"
+ },
+ {
+ "name": "Kioti 368",
+ "pos_x": -9531.125,
+ "pos_y": -910.4375,
+ "pos_z": 19776.1875,
+ "stations": "Kremmen's Respite"
+ },
+ {
+ "name": "Kipgen",
+ "pos_x": 149,
+ "pos_y": -105.96875,
+ "pos_z": -31.9375,
+ "stations": "Cherry Dock,Keyes Mine,Anderson Stop"
+ },
+ {
+ "name": "Kipgen Mu",
+ "pos_x": 62.21875,
+ "pos_y": 77.75,
+ "pos_z": 147.15625,
+ "stations": "Littlewood's Claim"
+ },
+ {
+ "name": "Kipgenik",
+ "pos_x": -82.0625,
+ "pos_y": 34.0625,
+ "pos_z": -123.625,
+ "stations": "Verrazzano Camp,Kirtley Survey,Capek Installation"
+ },
+ {
+ "name": "Kipgeninka",
+ "pos_x": 58.125,
+ "pos_y": -75.46875,
+ "pos_z": 72.25,
+ "stations": "Maanen Landing,Oberth Landing,Jordan Enterprise"
+ },
+ {
+ "name": "Kipgensi",
+ "pos_x": -98.5625,
+ "pos_y": 94.71875,
+ "pos_z": 75.875,
+ "stations": "Smith Platform,Huberath Beacon"
+ },
+ {
+ "name": "Kipsigi",
+ "pos_x": -130.875,
+ "pos_y": 29.40625,
+ "pos_z": -117.96875,
+ "stations": "Marley Terminal"
+ },
+ {
+ "name": "Kipsigines",
+ "pos_x": 47.75,
+ "pos_y": 30.3125,
+ "pos_z": 41.375,
+ "stations": "Sellers Hangar,Roggeveen Point"
+ },
+ {
+ "name": "Kiribalngu",
+ "pos_x": 80.65625,
+ "pos_y": 61.65625,
+ "pos_z": 13.65625,
+ "stations": "Kinsey Horizons"
+ },
+ {
+ "name": "Kiripibo",
+ "pos_x": 155.65625,
+ "pos_y": -28.40625,
+ "pos_z": -47.3125,
+ "stations": "Jones Horizons,Ross Station,Julian Bastion,Bloch Terminal"
+ },
+ {
+ "name": "Kirrae",
+ "pos_x": 112.46875,
+ "pos_y": -3.75,
+ "pos_z": 37.59375,
+ "stations": "Hedin Refinery,Kneale Hangar,Manakov Installation,Shaara Landing"
+ },
+ {
+ "name": "Kirram",
+ "pos_x": 133.4375,
+ "pos_y": 36.4375,
+ "pos_z": 30.59375,
+ "stations": "Disch Station,Walters' Progress,Barcelos City,Griffith Horizons"
+ },
+ {
+ "name": "Kisa",
+ "pos_x": 106.5,
+ "pos_y": -199.65625,
+ "pos_z": 104.6875,
+ "stations": "Thorne Vision,Lindstrand Vision,Keeler Installation"
+ },
+ {
+ "name": "Kisatsya",
+ "pos_x": -7.15625,
+ "pos_y": -157.09375,
+ "pos_z": 51.03125,
+ "stations": "Bohr Prospect,Giacconi Landing,Laird Plant"
+ },
+ {
+ "name": "Kisaywa",
+ "pos_x": -107.25,
+ "pos_y": -14.4375,
+ "pos_z": 29.15625,
+ "stations": "Gauss Mines,Kononenko Enterprise,Al-Farabi Colony"
+ },
+ {
+ "name": "Kishangumir",
+ "pos_x": -3.40625,
+ "pos_y": 17.3125,
+ "pos_z": -168.59375,
+ "stations": "Alten Laboratory,Hayden's Exile"
+ },
+ {
+ "name": "Kishijoten",
+ "pos_x": 76.59375,
+ "pos_y": 67.6875,
+ "pos_z": 105.03125,
+ "stations": "Tesla Depot,Yegorov Port,Shosuke's Inheritance"
+ },
+ {
+ "name": "Kishpakho",
+ "pos_x": -7.84375,
+ "pos_y": 97.125,
+ "pos_z": 76.90625,
+ "stations": "Crown City,Lanier Prospect"
+ },
+ {
+ "name": "Kisina",
+ "pos_x": 77.1875,
+ "pos_y": 18.5,
+ "pos_z": -94.28125,
+ "stations": "Oersted Stop,Worden Mines,Low Stop"
+ },
+ {
+ "name": "Kising",
+ "pos_x": 100.15625,
+ "pos_y": -260.8125,
+ "pos_z": 59.03125,
+ "stations": "Lambert Orbital,Hyakutake Enterprise,Swift Enterprise,Nesvadba's Inheritance"
+ },
+ {
+ "name": "Kisinga",
+ "pos_x": -4.40625,
+ "pos_y": -172.9375,
+ "pos_z": 97.6875,
+ "stations": "Slipher Reach,Pond Colony,Steinmuller Installation,Wolf Hub"
+ },
+ {
+ "name": "Kisir",
+ "pos_x": 126.09375,
+ "pos_y": -68.28125,
+ "pos_z": 106.75,
+ "stations": "Melotte Estate,Bickel Hub,Biermann Hub"
+ },
+ {
+ "name": "Kissensi",
+ "pos_x": 33.3125,
+ "pos_y": 84.90625,
+ "pos_z": -72.1875,
+ "stations": "Laplace Refinery,Smith Beacon"
+ },
+ {
+ "name": "Kissi",
+ "pos_x": -149.59375,
+ "pos_y": -33.28125,
+ "pos_z": -27.78125,
+ "stations": "Rzeppa Orbital"
+ },
+ {
+ "name": "Kissist",
+ "pos_x": -36.78125,
+ "pos_y": -91.125,
+ "pos_z": 19.25,
+ "stations": "Hauck Orbital"
+ },
+ {
+ "name": "Kistha",
+ "pos_x": 77.28125,
+ "pos_y": -30.59375,
+ "pos_z": -7.8125,
+ "stations": "Elion City,Davis Terminal,Velho Landing,Al-Khalili Plant"
+ },
+ {
+ "name": "Kistsi",
+ "pos_x": -97.8125,
+ "pos_y": -0.90625,
+ "pos_z": 119.3125,
+ "stations": "Revin Horizons,Korniyenko Station,Felice Penal colony,Berezovoy Station"
+ },
+ {
+ "name": "Kistultula",
+ "pos_x": 59.125,
+ "pos_y": 14.46875,
+ "pos_z": 109.9375,
+ "stations": "Wright Dock,Barry Station,Culpeper's Claim"
+ },
+ {
+ "name": "Kita Indji",
+ "pos_x": 96.78125,
+ "pos_y": -95.34375,
+ "pos_z": -60.375,
+ "stations": "Cerulli Platform,Kuttner Reach,Fokker Orbital,Seyfert Survey"
+ },
+ {
+ "name": "Kitae",
+ "pos_x": -13.5,
+ "pos_y": 24.75,
+ "pos_z": -56.46875,
+ "stations": "Bellamy Dock,al-Haytham Dock,Harness Survey"
+ },
+ {
+ "name": "Kitaki",
+ "pos_x": 31.84375,
+ "pos_y": -140.21875,
+ "pos_z": 95.25,
+ "stations": "Paola Vision,Joseph Delambre Hub,MacGill Hub,Hawker Lab,Back Vision"
+ },
+ {
+ "name": "Kitani",
+ "pos_x": 158.03125,
+ "pos_y": 8.03125,
+ "pos_z": 84.125,
+ "stations": "Barreiros Landing,Ing Port,Desargues Survey,Toll Orbital"
+ },
+ {
+ "name": "Kitara",
+ "pos_x": 6.5625,
+ "pos_y": 106.25,
+ "pos_z": -23,
+ "stations": "Russell Station"
+ },
+ {
+ "name": "Kitchang Mu",
+ "pos_x": 77.65625,
+ "pos_y": -16.875,
+ "pos_z": 135.25,
+ "stations": "Stefansson Station,Janes Port,Rustah Hub,Tayler Silo,Gelfand Arena"
+ },
+ {
+ "name": "Kitche",
+ "pos_x": -0.96875,
+ "pos_y": -116.5,
+ "pos_z": 39.53125,
+ "stations": "Myasishchev Ring,Brouwer Hub,Oosterhoff City"
+ },
+ {
+ "name": "Kitchih",
+ "pos_x": 66.03125,
+ "pos_y": -42.78125,
+ "pos_z": -90.21875,
+ "stations": "Paez Platform"
+ },
+ {
+ "name": "Kitchitatis",
+ "pos_x": -63.1875,
+ "pos_y": -92.28125,
+ "pos_z": 139.6875,
+ "stations": "Stanier Colony,Massimino Terminal,Kratman Terminal,Lerner Relay"
+ },
+ {
+ "name": "Kitchosat",
+ "pos_x": 21.90625,
+ "pos_y": 64.8125,
+ "pos_z": 107.625,
+ "stations": "Varthema City,Moresby Station,Ocampo Hub,Newcomen Survey"
+ },
+ {
+ "name": "Kites",
+ "pos_x": 27.78125,
+ "pos_y": -206.59375,
+ "pos_z": -43.75,
+ "stations": "Chertovsky Arena"
+ },
+ {
+ "name": "Kitja",
+ "pos_x": -103.25,
+ "pos_y": -2.125,
+ "pos_z": 156.40625,
+ "stations": "Sohl Prospect"
+ },
+ {
+ "name": "Kitjali",
+ "pos_x": 34.65625,
+ "pos_y": -108.875,
+ "pos_z": 54.90625,
+ "stations": "Jenkins Dock,Webb Station,Faye Barracks"
+ },
+ {
+ "name": "Kitjarundi",
+ "pos_x": 50.71875,
+ "pos_y": -101.8125,
+ "pos_z": -100.625,
+ "stations": "Ziegler Platform,Foerster Hangar,Armero Port,Moskowitz Works,Rawat Prospect"
+ },
+ {
+ "name": "Kivah",
+ "pos_x": 84.0625,
+ "pos_y": 1.28125,
+ "pos_z": 11.125,
+ "stations": "Sadi Carnot Ring,O'Donnell Exchange,So-yeon Terminal,Noguchi Orbital"
+ },
+ {
+ "name": "Kivas",
+ "pos_x": -101.40625,
+ "pos_y": 64.40625,
+ "pos_z": 121.84375,
+ "stations": "Edwards' Claim,Ross Stop,Lander Base"
+ },
+ {
+ "name": "Kivati",
+ "pos_x": 54.0625,
+ "pos_y": -201.9375,
+ "pos_z": 97.375,
+ "stations": "Herzog Depot"
+ },
+ {
+ "name": "Klamath",
+ "pos_x": 11.40625,
+ "pos_y": 38.625,
+ "pos_z": 93.3125,
+ "stations": "Dirac Station,Thoreau Orbital,Qurra Enterprise,Rozhdestvensky City"
+ },
+ {
+ "name": "Klikini",
+ "pos_x": 134.90625,
+ "pos_y": -23.0625,
+ "pos_z": 44.8125,
+ "stations": "Fulton Survey"
+ },
+ {
+ "name": "Klikis",
+ "pos_x": 38.28125,
+ "pos_y": 74.6875,
+ "pos_z": 163.71875,
+ "stations": "Baird Dock,Leinster Prospect,Shaw Dock,Baudin Dock,Ibold Relay,Robinson Observatory"
+ },
+ {
+ "name": "Klikudiya",
+ "pos_x": 32.4375,
+ "pos_y": 36.6875,
+ "pos_z": -69.4375,
+ "stations": "Arrhenius Orbital,Bresnik Terminal,Zalyotin Ring,Eanes Penal colony"
+ },
+ {
+ "name": "Klikumad",
+ "pos_x": 129.5625,
+ "pos_y": -12.75,
+ "pos_z": -40.125,
+ "stations": "Mitchell Works,Burbank Colony"
+ },
+ {
+ "name": "Klos",
+ "pos_x": -113.9375,
+ "pos_y": 57.65625,
+ "pos_z": 92.25,
+ "stations": "Tevis Outpost"
+ },
+ {
+ "name": "Knepagari",
+ "pos_x": 96.71875,
+ "pos_y": -141.28125,
+ "pos_z": -4.78125,
+ "stations": "Paczynski Platform"
+ },
+ {
+ "name": "Knouphinan",
+ "pos_x": 87.5625,
+ "pos_y": 10.78125,
+ "pos_z": -116.21875,
+ "stations": "Mawson Platform,Hinz's Claim,King Base"
+ },
+ {
+ "name": "Knumadi",
+ "pos_x": 97.21875,
+ "pos_y": -117.78125,
+ "pos_z": -57.5625,
+ "stations": "von Zach Station,Jones Horizons,Gregory Mine"
+ },
+ {
+ "name": "Knumclaw",
+ "pos_x": 150.46875,
+ "pos_y": -12.5,
+ "pos_z": 40.03125,
+ "stations": "Hipparchus Orbital,Anderson Enterprise,Gray Laboratory"
+ },
+ {
+ "name": "Koamu Yi",
+ "pos_x": -87.4375,
+ "pos_y": 119.09375,
+ "pos_z": -39.03125,
+ "stations": "Moore Dock,Spring Installation,Xiaoguan Landing,Fincke Colony"
+ },
+ {
+ "name": "Koamul",
+ "pos_x": -26.46875,
+ "pos_y": 24.90625,
+ "pos_z": -119.90625,
+ "stations": "Franklin Penal colony,Nikitin Prospect"
+ },
+ {
+ "name": "Koamulotri",
+ "pos_x": 158.625,
+ "pos_y": -77.78125,
+ "pos_z": 44.125,
+ "stations": "Giraud Settlement,Bradshaw Vision,Ulloa Terminal"
+ },
+ {
+ "name": "Koamutera",
+ "pos_x": -110.34375,
+ "pos_y": -44.625,
+ "pos_z": 56.34375,
+ "stations": "Sabine Dock,Nachtigal Beacon,Fibonacci Plant"
+ },
+ {
+ "name": "Koamuth",
+ "pos_x": -162.0625,
+ "pos_y": -34.90625,
+ "pos_z": -14.75,
+ "stations": "Lousma Works"
+ },
+ {
+ "name": "Koar",
+ "pos_x": 74.15625,
+ "pos_y": -198.21875,
+ "pos_z": 95.28125,
+ "stations": "Bolden Point,Curtis Works"
+ },
+ {
+ "name": "Koara",
+ "pos_x": -74.25,
+ "pos_y": 140.6875,
+ "pos_z": 24.59375,
+ "stations": "Killough Dock,Harding Gateway,Goulart Orbital,McHugh Settlement"
+ },
+ {
+ "name": "Koartoq",
+ "pos_x": 174.65625,
+ "pos_y": -203.96875,
+ "pos_z": -12.90625,
+ "stations": "Fiorilla Platform"
+ },
+ {
+ "name": "Kobog",
+ "pos_x": -31.90625,
+ "pos_y": 54.90625,
+ "pos_z": 112.15625,
+ "stations": "Francisco de Eliza Orbital,Siodmak Plant"
+ },
+ {
+ "name": "Koboga",
+ "pos_x": 167.03125,
+ "pos_y": -41.4375,
+ "pos_z": -26.1875,
+ "stations": "Brundage Dock"
+ },
+ {
+ "name": "Kobogdi",
+ "pos_x": 52.875,
+ "pos_y": -138.15625,
+ "pos_z": -120,
+ "stations": "Oramus Beacon"
+ },
+ {
+ "name": "Koboroma",
+ "pos_x": -3.5,
+ "pos_y": -18.96875,
+ "pos_z": 112.75,
+ "stations": "Thagard Vision,Jendrassik Point"
+ },
+ {
+ "name": "Kocab",
+ "pos_x": -91.78125,
+ "pos_y": 85.3125,
+ "pos_z": -38,
+ "stations": "Napier Dock,Drzewiecki Gateway,Kratman Holdings,Peirce Orbital,Wheelock Gateway"
+ },
+ {
+ "name": "Koch",
+ "pos_x": 8.4375,
+ "pos_y": -17.875,
+ "pos_z": -135.59375,
+ "stations": "Selberg Dock,Paez Dock,Nachtigal Landing"
+ },
+ {
+ "name": "Kocha",
+ "pos_x": 124.28125,
+ "pos_y": -68.21875,
+ "pos_z": 42.25,
+ "stations": "Slipher Arena"
+ },
+ {
+ "name": "Kochifuna",
+ "pos_x": 13.5,
+ "pos_y": 153.90625,
+ "pos_z": -2.6875,
+ "stations": "Lebesgue Terminal,Elvstrom Base,Smith Survey"
+ },
+ {
+ "name": "Kochil",
+ "pos_x": 99.9375,
+ "pos_y": 115.09375,
+ "pos_z": 52.8125,
+ "stations": "Drake Prospect,Martins Orbital,Aikin Settlement"
+ },
+ {
+ "name": "Kochole",
+ "pos_x": -83.25,
+ "pos_y": -47.1875,
+ "pos_z": 26.46875,
+ "stations": "Judson Terminal,Silverberg Ring,Bombelli Enterprise,Diophantus Landing,Cremona's Inheritance"
+ },
+ {
+ "name": "Koeng Pora",
+ "pos_x": 163.5625,
+ "pos_y": -198.375,
+ "pos_z": 74.5625,
+ "stations": "Block Survey,Dantec Settlement,Tuttle Vision,Frazetta Station"
+ },
+ {
+ "name": "Koenpa",
+ "pos_x": -73.25,
+ "pos_y": -94.125,
+ "pos_z": -121.84375,
+ "stations": "Bondar Vision,Hannu Hub"
+ },
+ {
+ "name": "Koenpeiadal",
+ "pos_x": 153.59375,
+ "pos_y": -243.5625,
+ "pos_z": 7.28125,
+ "stations": "Nilson Survey"
+ },
+ {
+ "name": "Kogich",
+ "pos_x": -13.9375,
+ "pos_y": -28.21875,
+ "pos_z": -82.84375,
+ "stations": "Williamson Hub"
+ },
+ {
+ "name": "Koine",
+ "pos_x": 107.40625,
+ "pos_y": -40.59375,
+ "pos_z": -71.5625,
+ "stations": "Viete Colony,Ibold Vision"
+ },
+ {
+ "name": "Koing",
+ "pos_x": 156.6875,
+ "pos_y": -220.59375,
+ "pos_z": 60.40625,
+ "stations": "Xin Mines,Banach's Progress"
+ },
+ {
+ "name": "Koira",
+ "pos_x": 137.0625,
+ "pos_y": 17.875,
+ "pos_z": 52.625,
+ "stations": "Ahern Installation"
+ },
+ {
+ "name": "Koirada",
+ "pos_x": 134.34375,
+ "pos_y": -19.90625,
+ "pos_z": -1,
+ "stations": "Bobko Station,Sellings Landing,Gooch Orbital"
+ },
+ {
+ "name": "Koirao",
+ "pos_x": 155.53125,
+ "pos_y": 50.09375,
+ "pos_z": -31.03125,
+ "stations": "Kuhn City,Tyurin Ring,Casper Terminal,Thornycroft Beacon"
+ },
+ {
+ "name": "Koiri",
+ "pos_x": -28.125,
+ "pos_y": -161.03125,
+ "pos_z": -44.65625,
+ "stations": "Koishikawa Dock,Dubyago Dock,Chelomey Port"
+ },
+ {
+ "name": "Koirisas",
+ "pos_x": 154,
+ "pos_y": -75.125,
+ "pos_z": 26.5625,
+ "stations": "Walotsky Stop,Loewy Depot"
+ },
+ {
+ "name": "Kojeara",
+ "pos_x": -9513.09375,
+ "pos_y": -908.84375,
+ "pos_z": 19814.28125,
+ "stations": "TolaGarf's Junkyard,Oswald Park"
+ },
+ {
+ "name": "Kojin",
+ "pos_x": 16.15625,
+ "pos_y": -68.65625,
+ "pos_z": 87.8125,
+ "stations": "Delany Freeport,Harness Depot"
+ },
+ {
+ "name": "Kojinbaia",
+ "pos_x": 135.5625,
+ "pos_y": -117.5,
+ "pos_z": 29.875,
+ "stations": "Phillips Landing,Theodor Winnecke Orbital,la Cosa Point"
+ },
+ {
+ "name": "Kokaana",
+ "pos_x": -140.65625,
+ "pos_y": 20.78125,
+ "pos_z": 27.96875,
+ "stations": "Planck Port,Gerst Hub,Johnson Settlement,Rawn's Claim,Makarov Market"
+ },
+ {
+ "name": "Kokaangin",
+ "pos_x": 69.5625,
+ "pos_y": -221.65625,
+ "pos_z": 72.8125,
+ "stations": "Korolev Terminal,Lindblad Gateway,Carnera Vision,Hippalus Penal colony,Sugano Beacon"
+ },
+ {
+ "name": "Kokan",
+ "pos_x": -20.1875,
+ "pos_y": 115.3125,
+ "pos_z": 78.25,
+ "stations": "Citroen Colony"
+ },
+ {
+ "name": "Kokana",
+ "pos_x": -55.125,
+ "pos_y": 60.84375,
+ "pos_z": 131.53125,
+ "stations": "Ali Port,Anders Mines"
+ },
+ {
+ "name": "Kokanapus",
+ "pos_x": 144.40625,
+ "pos_y": -39,
+ "pos_z": -56.34375,
+ "stations": "Sadi Carnot Dock,Mukai Base"
+ },
+ {
+ "name": "Kokap",
+ "pos_x": 112.625,
+ "pos_y": -204.84375,
+ "pos_z": 11.03125,
+ "stations": "Royo Stop"
+ },
+ {
+ "name": "Kokara",
+ "pos_x": 17.15625,
+ "pos_y": 118,
+ "pos_z": 42.15625,
+ "stations": "Haller Port,Weil Enterprise,Ehrlich Point"
+ },
+ {
+ "name": "Kokarwang",
+ "pos_x": 93.125,
+ "pos_y": 29.4375,
+ "pos_z": -103.09375,
+ "stations": "Sturckow Port,Wetherbee Port,Hutchinson Settlement"
+ },
+ {
+ "name": "Kokary",
+ "pos_x": 3.5,
+ "pos_y": -10.3125,
+ "pos_z": -11.4375,
+ "stations": "Foda's Inheritance,Gidzenko Terminal,Cori Gateway,Faraday Enterprise,Treshchov Point"
+ },
+ {
+ "name": "Kokatese",
+ "pos_x": 66.9375,
+ "pos_y": -32.34375,
+ "pos_z": -34.96875,
+ "stations": "Koontz Enterprise,Goldstein Settlement,Chorel Vision,Humphreys Plant"
+ },
+ {
+ "name": "Kokipik",
+ "pos_x": 88.125,
+ "pos_y": 15.40625,
+ "pos_z": 20.09375,
+ "stations": "Clifford Refinery,Laveykin Plant,Grijalva Point"
+ },
+ {
+ "name": "Koknan",
+ "pos_x": -12.9375,
+ "pos_y": -181.15625,
+ "pos_z": 95.96875,
+ "stations": "Moffat Terminal,Brooks Prospect,Herbig Holdings,Baydukov Enterprise"
+ },
+ {
+ "name": "Koko",
+ "pos_x": 98.15625,
+ "pos_y": -164.6875,
+ "pos_z": 160.53125,
+ "stations": "MacGill Terminal,Hipparchus Settlement,Crown Oasis"
+ },
+ {
+ "name": "Koko Oh",
+ "pos_x": -139.875,
+ "pos_y": -37.21875,
+ "pos_z": -35.875,
+ "stations": "Finch Reach,Stillman Dock,Harness Legacy"
+ },
+ {
+ "name": "Kokobi",
+ "pos_x": 59.15625,
+ "pos_y": 116.40625,
+ "pos_z": 27.5625,
+ "stations": "Lenthall Freeport,Stefanyshyn-Piper Barracks"
+ },
+ {
+ "name": "Kokobididji",
+ "pos_x": 102.1875,
+ "pos_y": -159.5,
+ "pos_z": 145.9375,
+ "stations": "Sheremetevsky Vision,Kirby Port"
+ },
+ {
+ "name": "Kokobii",
+ "pos_x": 83.59375,
+ "pos_y": -69.46875,
+ "pos_z": -9.78125,
+ "stations": "Bauman Station,Martins Installation,Consolmagno Keep,Kranz Gateway,Filter Reach,Anderson Hub"
+ },
+ {
+ "name": "Kokoi",
+ "pos_x": 62.5625,
+ "pos_y": 98.5625,
+ "pos_z": -34.96875,
+ "stations": "Chun Stop,Stafford Vista"
+ },
+ {
+ "name": "Kokoimudji",
+ "pos_x": 69.3125,
+ "pos_y": 18.125,
+ "pos_z": 98.40625,
+ "stations": "Siodmak Station,Sternberg Settlement,Tem Port,Quinn Orbital,Auld Plant"
+ },
+ {
+ "name": "Kokoja",
+ "pos_x": 182.375,
+ "pos_y": -169.0625,
+ "pos_z": 43.21875,
+ "stations": "Miller Horizons,Ahnert Dock,Kingsbury Settlement,Jones Vision"
+ },
+ {
+ "name": "Kokojas",
+ "pos_x": -83.0625,
+ "pos_y": -41.46875,
+ "pos_z": 40.875,
+ "stations": "Mendel Survey"
+ },
+ {
+ "name": "Kokojawa",
+ "pos_x": -34.40625,
+ "pos_y": -35.09375,
+ "pos_z": 104.0625,
+ "stations": "Tayler Enterprise,Griffin Plant,Fraser Terminal,Jordan Gateway,Budrys Colony"
+ },
+ {
+ "name": "Kokojelatia",
+ "pos_x": -117.90625,
+ "pos_y": -124.5,
+ "pos_z": -3.125,
+ "stations": "Lie Orbital,Semeonis Dock,Sheckley Settlement"
+ },
+ {
+ "name": "Kokojina",
+ "pos_x": -5.25,
+ "pos_y": -6.90625,
+ "pos_z": 56.53125,
+ "stations": "Ali Ring,Kinsey Terminal"
+ },
+ {
+ "name": "Kokokomi",
+ "pos_x": 28.03125,
+ "pos_y": -22.09375,
+ "pos_z": 83.34375,
+ "stations": "Smith Enterprise,Kummer Beacon,Stasheff Vista"
+ },
+ {
+ "name": "Kokoku",
+ "pos_x": 100.3125,
+ "pos_y": -96.625,
+ "pos_z": 102.9375,
+ "stations": "Abbot Gateway,Ziegel Vision,Osterbrock Ring,Markham Depot"
+ },
+ {
+ "name": "Kokoller",
+ "pos_x": -23.5625,
+ "pos_y": -15.21875,
+ "pos_z": 102.875,
+ "stations": "Utley Hub,Blodgett's Folly,Wandrei Hub,Bessemer Installation,Cadamosto Dock"
+ },
+ {
+ "name": "Kokomi",
+ "pos_x": 35,
+ "pos_y": -30.5,
+ "pos_z": -73.0625,
+ "stations": "Ricardo Orbital"
+ },
+ {
+ "name": "Kokomini",
+ "pos_x": 82.3125,
+ "pos_y": 68.5,
+ "pos_z": 115.71875,
+ "stations": "Anthony Installation,Elder Port,Filter Observatory"
+ },
+ {
+ "name": "Kokomisii",
+ "pos_x": 88.6875,
+ "pos_y": -199,
+ "pos_z": 117.28125,
+ "stations": "Hill Tinsley Dock,Minkowski Landing,Corbusier Station"
+ },
+ {
+ "name": "Kokonda",
+ "pos_x": 163.875,
+ "pos_y": -105.40625,
+ "pos_z": 28.6875,
+ "stations": "Sandage Landing,Pond Orbital,Goryu Vision"
+ },
+ {
+ "name": "Kokondii",
+ "pos_x": -111.84375,
+ "pos_y": 10.375,
+ "pos_z": 154.625,
+ "stations": "Barcelo Terminal,Darboux Terminal,Bounds Laboratory,Bokeili Mines,Wallace Hub"
+ },
+ {
+ "name": "Kokondji",
+ "pos_x": 161.15625,
+ "pos_y": -242.625,
+ "pos_z": 59.28125,
+ "stations": "Chretien Oudemans Landing,Cerulli Enterprise,Whitford Landing,Bellamy Keep"
+ },
+ {
+ "name": "Kokong Hsin",
+ "pos_x": 50.09375,
+ "pos_y": -78.75,
+ "pos_z": 135.21875,
+ "stations": "Niemeyer Colony,Kempf Port,Bode Colony,Filter Keep,Tucker Base"
+ },
+ {
+ "name": "Kokongyi",
+ "pos_x": 154.96875,
+ "pos_y": -23.75,
+ "pos_z": 72,
+ "stations": "Baker Landing,Jordan's Claim,Chaviano Refinery"
+ },
+ {
+ "name": "Kokonjekodi",
+ "pos_x": 109.21875,
+ "pos_y": -142.5625,
+ "pos_z": 2.125,
+ "stations": "Ulloa Vision,Trujillo Dock,Kushida Refinery,Naboth Dock,Keyes Prospect"
+ },
+ {
+ "name": "Kokopatji",
+ "pos_x": 152.34375,
+ "pos_y": -45.71875,
+ "pos_z": 20.5625,
+ "stations": "Keeler Horizons,Schmidt Lab"
+ },
+ {
+ "name": "Kokopatun",
+ "pos_x": -38.78125,
+ "pos_y": -63.5,
+ "pos_z": 150.125,
+ "stations": "Oltion's Claim"
+ },
+ {
+ "name": "Kokosh",
+ "pos_x": 116.125,
+ "pos_y": 55.34375,
+ "pos_z": 78.46875,
+ "stations": "Preuss' Claim"
+ },
+ {
+ "name": "Kokoto",
+ "pos_x": 39.15625,
+ "pos_y": 40.5,
+ "pos_z": 28,
+ "stations": "Pogue Hub,Bester Silo,Crown Settlement,Herrington City"
+ },
+ {
+ "name": "Kokowar",
+ "pos_x": 4.65625,
+ "pos_y": -101.9375,
+ "pos_z": 18.46875,
+ "stations": "Karman Ring,Rowley Landing,Suydam Gateway,Babakin's Inheritance,Weierstrass Keep"
+ },
+ {
+ "name": "Kokowatsu",
+ "pos_x": -5.59375,
+ "pos_y": -224.28125,
+ "pos_z": -1.15625,
+ "stations": "Fischer Landing,Aoki Keep"
+ },
+ {
+ "name": "Kokyambi",
+ "pos_x": -66.46875,
+ "pos_y": -104.21875,
+ "pos_z": -21.8125,
+ "stations": "Pook Reformatory,Macdonald Outpost,Morris Beacon"
+ },
+ {
+ "name": "Kol",
+ "pos_x": -21.3125,
+ "pos_y": -101.5625,
+ "pos_z": -142.34375,
+ "stations": "Person Legacy"
+ },
+ {
+ "name": "Kolabiety",
+ "pos_x": -34.59375,
+ "pos_y": -34.4375,
+ "pos_z": -152.75,
+ "stations": "Melroy Vision"
+ },
+ {
+ "name": "Kolabinates",
+ "pos_x": -100.125,
+ "pos_y": -99.625,
+ "pos_z": 18.625,
+ "stations": "Grant Plant"
+ },
+ {
+ "name": "Kolaga",
+ "pos_x": -32.9375,
+ "pos_y": -14.21875,
+ "pos_z": -97.46875,
+ "stations": "Britnev Hub,Clapperton Station,Taylor Relay,Wiener Landing"
+ },
+ {
+ "name": "Kolandira",
+ "pos_x": 128.46875,
+ "pos_y": -41.8125,
+ "pos_z": 12.125,
+ "stations": "Wilson Penal colony,Schouten Vision"
+ },
+ {
+ "name": "Kolanji",
+ "pos_x": -81.59375,
+ "pos_y": 18.3125,
+ "pos_z": 20.09375,
+ "stations": "Scott Station,McDivitt Base,Lucretius Port,Hirase Ring,Fadlan Arsenal"
+ },
+ {
+ "name": "Kolapa",
+ "pos_x": -25.125,
+ "pos_y": -120.84375,
+ "pos_z": 31.75,
+ "stations": "Thesiger Silo,Parkinson Landing,Roemer Installation,Koolhaas Hub,Joy Refinery"
+ },
+ {
+ "name": "Koleti",
+ "pos_x": 118.84375,
+ "pos_y": -70.3125,
+ "pos_z": -20.53125,
+ "stations": "Pannekoek Dock,Brom Ring,Harrison Port,Galle Station,Celebi Survey,Chiang Orbital,Borrelly City,Antoniadi City,Vasquez de Coronado Plant"
+ },
+ {
+ "name": "Kolewixil",
+ "pos_x": 128.28125,
+ "pos_y": -93.21875,
+ "pos_z": -75.21875,
+ "stations": "Nicholson Settlement"
+ },
+ {
+ "name": "Kolhou Wu",
+ "pos_x": 37.84375,
+ "pos_y": -222.5,
+ "pos_z": -41.28125,
+ "stations": "Zaschka Mines"
+ },
+ {
+ "name": "Kolhyni",
+ "pos_x": 50.40625,
+ "pos_y": -42.59375,
+ "pos_z": -79,
+ "stations": "Soddy Station"
+ },
+ {
+ "name": "Kolhynichi",
+ "pos_x": -142.625,
+ "pos_y": 5.625,
+ "pos_z": -105.96875,
+ "stations": "Hassanein City,Hovgaard Orbital,Shiras Gateway,Pinto Bastion"
+ },
+ {
+ "name": "Koli Discii",
+ "pos_x": 108.84375,
+ "pos_y": 83.53125,
+ "pos_z": -58.71875,
+ "stations": "Gerlache Base,MacLean Terminal,Pennington Enterprise,Vishweswarayya Orbital,He Works"
+ },
+ {
+ "name": "Kolibalita",
+ "pos_x": -1.09375,
+ "pos_y": 139.46875,
+ "pos_z": -64.40625,
+ "stations": "Baturin Port"
+ },
+ {
+ "name": "Kolingo",
+ "pos_x": 156.28125,
+ "pos_y": -56.375,
+ "pos_z": 33.0625,
+ "stations": "Faber Survey,Pratchett Camp,Allen St. John Platform"
+ },
+ {
+ "name": "Kolitja",
+ "pos_x": 5.21875,
+ "pos_y": -28.75,
+ "pos_z": -69.875,
+ "stations": "Puleston Point,Nakaya Station"
+ },
+ {
+ "name": "Koljak",
+ "pos_x": 27.875,
+ "pos_y": -19.4375,
+ "pos_z": -149.625,
+ "stations": "Bierce Dock,Clayton Survey"
+ },
+ {
+ "name": "Koljakinna",
+ "pos_x": 102.625,
+ "pos_y": 132.53125,
+ "pos_z": -41.59375,
+ "stations": "Tall Orbital"
+ },
+ {
+ "name": "Koljankirna",
+ "pos_x": 3.46875,
+ "pos_y": 118.15625,
+ "pos_z": 32,
+ "stations": "Jendrassik Vision,Rzeppa Vision,Nye Terminal,Slusser's Progress"
+ },
+ {
+ "name": "Koller",
+ "pos_x": 83.34375,
+ "pos_y": 18.40625,
+ "pos_z": 99.3125,
+ "stations": "Mitropoulos Colony,Xuanzang Dock,Cummings Platform,Hawke Camp,Nobel Landing"
+ },
+ {
+ "name": "Kollobokos",
+ "pos_x": -78.84375,
+ "pos_y": -3.9375,
+ "pos_z": 31.84375,
+ "stations": "Agassiz Hub,Fisher Settlement"
+ },
+ {
+ "name": "Kolyan",
+ "pos_x": -106.78125,
+ "pos_y": -32.625,
+ "pos_z": -50.90625,
+ "stations": "Scortia Ring,Wilson Horizons,Clough's Pride"
+ },
+ {
+ "name": "Kolyawa",
+ "pos_x": -75.09375,
+ "pos_y": -31.84375,
+ "pos_z": -34.09375,
+ "stations": "Remec Gateway"
+ },
+ {
+ "name": "Komindjin",
+ "pos_x": -95.46875,
+ "pos_y": -2.3125,
+ "pos_z": 116.25,
+ "stations": "Nye Depot"
+ },
+ {
+ "name": "Komotae",
+ "pos_x": 24.25,
+ "pos_y": 83.90625,
+ "pos_z": -17.28125,
+ "stations": "Bluford Station,Morey Barracks,Feustel Park,Filipchenko Terminal"
+ },
+ {
+ "name": "Komovoy",
+ "pos_x": 70.03125,
+ "pos_y": 30.53125,
+ "pos_z": 44.15625,
+ "stations": "Silves Dock,Garnier Platform,Gentle Dock,Watson's Progress,Gell-Mann Barracks"
+ },
+ {
+ "name": "Konbudo",
+ "pos_x": 31.625,
+ "pos_y": -6.75,
+ "pos_z": 171.09375,
+ "stations": "Pook Depot"
+ },
+ {
+ "name": "Konburo",
+ "pos_x": 151.1875,
+ "pos_y": -32.375,
+ "pos_z": 34.8125,
+ "stations": "Kludze Port"
+ },
+ {
+ "name": "Konburus",
+ "pos_x": -111.90625,
+ "pos_y": 14.125,
+ "pos_z": 147.78125,
+ "stations": "Kornbluth Horizons,O'Leary Bastion,Levi-Civita Ring"
+ },
+ {
+ "name": "Kondadora",
+ "pos_x": 25.40625,
+ "pos_y": -75.4375,
+ "pos_z": -57.125,
+ "stations": "Rennie Enterprise,Smith Station,Nikolayev Station,Harding Base,Haignere Installation"
+ },
+ {
+ "name": "Kondareni",
+ "pos_x": 24.4375,
+ "pos_y": -259.71875,
+ "pos_z": -19.875,
+ "stations": "Dillon Relay"
+ },
+ {
+ "name": "Kondon",
+ "pos_x": 69.4375,
+ "pos_y": -1.625,
+ "pos_z": -85.53125,
+ "stations": "Atwood Relay,Ziljak Silo"
+ },
+ {
+ "name": "Kondonner",
+ "pos_x": 11.9375,
+ "pos_y": -109.59375,
+ "pos_z": 134.3125,
+ "stations": "Clark Vision,Fabricius Station,Gentil Dock,Matthews Base"
+ },
+ {
+ "name": "Kondoquit",
+ "pos_x": 95.875,
+ "pos_y": -221.6875,
+ "pos_z": 38.71875,
+ "stations": "Perlmutter Dock,RenenBellot Settlement,Korolev Laboratory,Naddoddur Barracks,McDevitt Prospect"
+ },
+ {
+ "name": "Kondovii",
+ "pos_x": -117.375,
+ "pos_y": 23.1875,
+ "pos_z": -6.6875,
+ "stations": "Schottky Port,Schlegel Port,Kubasov Beacon"
+ },
+ {
+ "name": "Kondriates",
+ "pos_x": -86.9375,
+ "pos_y": 94.3125,
+ "pos_z": -92.09375,
+ "stations": "Polya Mines,Hand Survey"
+ },
+ {
+ "name": "Kondrustem",
+ "pos_x": 20.78125,
+ "pos_y": -158.625,
+ "pos_z": 116.03125,
+ "stations": "Adkins Hub,Rondon Works,Peano Hub,Marsden Orbital,Arzachel Hub"
+ },
+ {
+ "name": "Konduwa",
+ "pos_x": -52.40625,
+ "pos_y": 107.84375,
+ "pos_z": 15.53125,
+ "stations": "Vance Station,Coulomb Hub,Felice Station,Narvaez Station,Bartlett Terminal,Kronecker Keep,Green's Progress,Lanier Legacy,Stasheff Keep,Malocello Forum"
+ },
+ {
+ "name": "Konejandi",
+ "pos_x": -14.96875,
+ "pos_y": -19.375,
+ "pos_z": -128.5,
+ "stations": "Andree Arena"
+ },
+ {
+ "name": "Konejang",
+ "pos_x": 87.71875,
+ "pos_y": -257.875,
+ "pos_z": 51.4375,
+ "stations": "Haro Relay,Roemer Dock"
+ },
+ {
+ "name": "Koney",
+ "pos_x": 104.3125,
+ "pos_y": 16.875,
+ "pos_z": -86.4375,
+ "stations": "McMahon Point,Smith Point"
+ },
+ {
+ "name": "Kong",
+ "pos_x": -93.21875,
+ "pos_y": -37.21875,
+ "pos_z": -120.15625,
+ "stations": "Pond Orbital,Griffith Plant,Morgan Depot"
+ },
+ {
+ "name": "Kong Dan",
+ "pos_x": 63.875,
+ "pos_y": -129,
+ "pos_z": -32.28125,
+ "stations": "Reber City,Brunner Port,Kelly Enterprise"
+ },
+ {
+ "name": "Kong Tzu",
+ "pos_x": 120.1875,
+ "pos_y": -42.9375,
+ "pos_z": 14.75,
+ "stations": "Ryazanski Ring,Slayton Installation,Turtledove Landing,Chilton Silo"
+ },
+ {
+ "name": "Kongaguan",
+ "pos_x": 15.96875,
+ "pos_y": -100.875,
+ "pos_z": -64.21875,
+ "stations": "Cixin Camp,Herreshoff Platform,Shapiro Settlement"
+ },
+ {
+ "name": "Kongan",
+ "pos_x": 101.8125,
+ "pos_y": -94.125,
+ "pos_z": -16.75,
+ "stations": "Miller Vision,Coelho Oasis,Atiyah Relay,Coddington Mines"
+ },
+ {
+ "name": "Kongga",
+ "pos_x": -104.15625,
+ "pos_y": 82.71875,
+ "pos_z": -32.375,
+ "stations": "Laplace Ring"
+ },
+ {
+ "name": "Konggal",
+ "pos_x": -96.4375,
+ "pos_y": 8.03125,
+ "pos_z": -93.46875,
+ "stations": "Brunel Prospect,Volta Landing"
+ },
+ {
+ "name": "Konggari",
+ "pos_x": 37,
+ "pos_y": -135.09375,
+ "pos_z": -79,
+ "stations": "Kent Prospect,Cook Colony"
+ },
+ {
+ "name": "Konggul",
+ "pos_x": 99.1875,
+ "pos_y": -1.25,
+ "pos_z": 49.75,
+ "stations": "Everest Prospect,Soukup Depot"
+ },
+ {
+ "name": "Kongi",
+ "pos_x": -149.21875,
+ "pos_y": 14.25,
+ "pos_z": -45.125,
+ "stations": "Springer Retreat"
+ },
+ {
+ "name": "Kongkhulym",
+ "pos_x": -15.9375,
+ "pos_y": -124.125,
+ "pos_z": 123.5625,
+ "stations": "Loewy Vision,Covington City"
+ },
+ {
+ "name": "Kongkomisso",
+ "pos_x": -40.25,
+ "pos_y": -58,
+ "pos_z": 39.4375,
+ "stations": "Glenn Horizons,McMullen Keep,McKay Enterprise"
+ },
+ {
+ "name": "Kongleri",
+ "pos_x": -85.71875,
+ "pos_y": 1.3125,
+ "pos_z": -144.5,
+ "stations": "Shatalov Platform,Boulton Installation,Chapman Dock"
+ },
+ {
+ "name": "Kongo",
+ "pos_x": 58.8125,
+ "pos_y": -79.625,
+ "pos_z": 87.96875,
+ "stations": "Thomson Survey,Molchanov Port"
+ },
+ {
+ "name": "Kongoloca",
+ "pos_x": -97.65625,
+ "pos_y": -29.21875,
+ "pos_z": 88.90625,
+ "stations": "Resnik Ring"
+ },
+ {
+ "name": "Kongwa",
+ "pos_x": 88.71875,
+ "pos_y": 68.46875,
+ "pos_z": 9.90625,
+ "stations": "Weiss Dock"
+ },
+ {
+ "name": "Konohavas",
+ "pos_x": 56.71875,
+ "pos_y": -199.25,
+ "pos_z": 116.34375,
+ "stations": "Theodor Winnecke Mines,Schommer Mine"
+ },
+ {
+ "name": "Konohi",
+ "pos_x": 16,
+ "pos_y": -53,
+ "pos_z": 102,
+ "stations": "Kuchner Refinery,Pavlou Hub"
+ },
+ {
+ "name": "Konorubang",
+ "pos_x": -119.1875,
+ "pos_y": -98.59375,
+ "pos_z": -83.6875,
+ "stations": "Abe Platform,McHugh's Progress"
+ },
+ {
+ "name": "Konoto",
+ "pos_x": 96.90625,
+ "pos_y": -245.90625,
+ "pos_z": 22.21875,
+ "stations": "Sopwith Prospect,Jeans Vision,Proctor Colony,Shelley's Claim,Macgregor Vision"
+ },
+ {
+ "name": "Kons",
+ "pos_x": 18.15625,
+ "pos_y": 100.90625,
+ "pos_z": -40.21875,
+ "stations": "Makarov Station,Farmer Port,Petaja Enterprise,Bradley Hub,Carrasco Landing"
+ },
+ {
+ "name": "Konsaxa",
+ "pos_x": -139.5,
+ "pos_y": 13.6875,
+ "pos_z": 73.71875,
+ "stations": "Adams Ring,Brackett Arsenal,Tilley Vision"
+ },
+ {
+ "name": "Konsu",
+ "pos_x": -127.375,
+ "pos_y": -69.78125,
+ "pos_z": 1.40625,
+ "stations": "Ham Colony,Feustel Beacon,Lind Terminal"
+ },
+ {
+ "name": "Kopernik",
+ "pos_x": -9504.75,
+ "pos_y": -901.75,
+ "pos_z": 19801.875,
+ "stations": "Kolonia Sobieski"
+ },
+ {
+ "name": "Kora Pin",
+ "pos_x": -41.125,
+ "pos_y": -107.75,
+ "pos_z": 29.15625,
+ "stations": "Copernicus Hub,Zamka Hub,Vesalius' Folly,Hilmers Dock,Yurchikhin Hub,Yamazaki Station"
+ },
+ {
+ "name": "Koraga",
+ "pos_x": -77.4375,
+ "pos_y": -22.375,
+ "pos_z": -33.125,
+ "stations": "Birkeland Depot,Pirsan Settlement,Baker Refinery"
+ },
+ {
+ "name": "Korai",
+ "pos_x": -164.4375,
+ "pos_y": 70.09375,
+ "pos_z": -25.6875,
+ "stations": "Gagnan Settlement,Budrys Platform,Herodotus Mine"
+ },
+ {
+ "name": "Koraik",
+ "pos_x": -29.875,
+ "pos_y": 57.375,
+ "pos_z": 41.40625,
+ "stations": "Barba Orbital,Locke Works"
+ },
+ {
+ "name": "Koraj",
+ "pos_x": -33.8125,
+ "pos_y": -17.21875,
+ "pos_z": -127.875,
+ "stations": "Leonov Terminal,England Terminal"
+ },
+ {
+ "name": "Korazotz",
+ "pos_x": -58.875,
+ "pos_y": -23.03125,
+ "pos_z": 74.75,
+ "stations": "Delbruck Mines,Nikolayev Platform"
+ },
+ {
+ "name": "Koren",
+ "pos_x": -114.03125,
+ "pos_y": -32.46875,
+ "pos_z": 95.03125,
+ "stations": "Davis Relay,Mackenzie Vision"
+ },
+ {
+ "name": "Korenses",
+ "pos_x": 126.75,
+ "pos_y": -14.125,
+ "pos_z": 46.375,
+ "stations": "Kwolek Hangar"
+ },
+ {
+ "name": "Koria",
+ "pos_x": -2.875,
+ "pos_y": 42.875,
+ "pos_z": -181,
+ "stations": "Gernsback Bastion,Green Prospect,Liouville Prospect"
+ },
+ {
+ "name": "Kormt",
+ "pos_x": -52.875,
+ "pos_y": -21.71875,
+ "pos_z": 84.65625,
+ "stations": "Perrin Terminal,MacLeod Base,Maudslay Hub"
+ },
+ {
+ "name": "Kornephoros",
+ "pos_x": -67.5,
+ "pos_y": 88.875,
+ "pos_z": 83.09375,
+ "stations": "Worden Station,Reilly Port,Hewish Enterprise,Makarov Port,Newcomen Dock,Stott Gateway,Northrop Port,Zhen Beacon"
+ },
+ {
+ "name": "Korotep",
+ "pos_x": -149.40625,
+ "pos_y": -31.03125,
+ "pos_z": 19.8125,
+ "stations": "Lopez de Haro Horizons,Crichton Horizons,Balmer Keep,Lem Vision"
+ },
+ {
+ "name": "Korovii",
+ "pos_x": -7.53125,
+ "pos_y": -17.21875,
+ "pos_z": 46.28125,
+ "stations": "Casper Ring,Dupuy de Lome Vision,Moore Gateway,Clervoy Gateway,England Terminal,Redi Gateway,Tryggvason Stop,Aleksandrov Orbital,McCarthy Enterprise,Stith Bastion"
+ },
+ {
+ "name": "Korring",
+ "pos_x": 24.5,
+ "pos_y": 99.5625,
+ "pos_z": 58.71875,
+ "stations": "Schiltberger Plant,Vespucci Refinery,Barbosa Prospect"
+ },
+ {
+ "name": "Korro Kung",
+ "pos_x": 81.03125,
+ "pos_y": 52.84375,
+ "pos_z": 31.5,
+ "stations": "Lonchakov Orbital,DeLucas City,Hadfield Hub"
+ },
+ {
+ "name": "Korrotra",
+ "pos_x": 147.8125,
+ "pos_y": -162.78125,
+ "pos_z": 6.4375,
+ "stations": "Young Arena"
+ },
+ {
+ "name": "Korubu",
+ "pos_x": -86.53125,
+ "pos_y": 39.0625,
+ "pos_z": -8.78125,
+ "stations": "Betancourt Terminal,Fuca Ring,Bailey Station,Birmingham Silo"
+ },
+ {
+ "name": "Korubures",
+ "pos_x": 115.15625,
+ "pos_y": -159.03125,
+ "pos_z": 96.25,
+ "stations": "Campbell Vision"
+ },
+ {
+ "name": "Korwali",
+ "pos_x": -113.21875,
+ "pos_y": -59.84375,
+ "pos_z": -7.5,
+ "stations": "Poncelet Freeport"
+ },
+ {
+ "name": "Korwei",
+ "pos_x": -31.25,
+ "pos_y": 1.78125,
+ "pos_z": 7.90625,
+ "stations": "Bernoulli Gateway,Wingqvist Enterprise,Barcelo Keep,Hartsfield Landing,Levi-Montalcini Observatory"
+ },
+ {
+ "name": "Koryaks",
+ "pos_x": 83.59375,
+ "pos_y": 25.28125,
+ "pos_z": -2.1875,
+ "stations": "Khrenov Outpost,Eskridge Vision"
+ },
+ {
+ "name": "Kosche",
+ "pos_x": -48.6875,
+ "pos_y": -30.03125,
+ "pos_z": 122.75,
+ "stations": "Litke Settlement,Gann City,Laing Survey,Schachner Enterprise,Meyrink's Inheritance"
+ },
+ {
+ "name": "Kosching",
+ "pos_x": -62.375,
+ "pos_y": -81.28125,
+ "pos_z": -42,
+ "stations": "Xing Station"
+ },
+ {
+ "name": "Kosena",
+ "pos_x": -40.40625,
+ "pos_y": 160.375,
+ "pos_z": -48.875,
+ "stations": "Gwynn Enterprise,Holub Landing"
+ },
+ {
+ "name": "Koseno",
+ "pos_x": 86.4375,
+ "pos_y": -47.59375,
+ "pos_z": 133.53125,
+ "stations": "Houten-Groeneveld Landing,Peltier Colony"
+ },
+ {
+ "name": "Kosensei",
+ "pos_x": 155.5,
+ "pos_y": -147.6875,
+ "pos_z": 15.125,
+ "stations": "Parker's Claim,Bouvard Reach"
+ },
+ {
+ "name": "Kosensi",
+ "pos_x": 16.8125,
+ "pos_y": 75.09375,
+ "pos_z": 138.84375,
+ "stations": "Remek Colony,Kraepelin Enterprise,Brand Prospect"
+ },
+ {
+ "name": "Kosha",
+ "pos_x": 146.4375,
+ "pos_y": -67.71875,
+ "pos_z": 6.75,
+ "stations": "Dufay Port,Heinlein Base,Tully Installation"
+ },
+ {
+ "name": "Koshans",
+ "pos_x": 138.4375,
+ "pos_y": -71.15625,
+ "pos_z": -9.8125,
+ "stations": "Burnham Installation,Secchi Dock"
+ },
+ {
+ "name": "Koshas",
+ "pos_x": 82.125,
+ "pos_y": -48.4375,
+ "pos_z": 110.8125,
+ "stations": "Rey Platform,Zelazny Colony"
+ },
+ {
+ "name": "Koshe",
+ "pos_x": 104.84375,
+ "pos_y": -8.1875,
+ "pos_z": -73.0625,
+ "stations": "Kavandi Port"
+ },
+ {
+ "name": "Koskit",
+ "pos_x": -71.96875,
+ "pos_y": -2.15625,
+ "pos_z": 48.78125,
+ "stations": "Meucci Survey,Oliver's Inheritance,Haberlandt Depot"
+ },
+ {
+ "name": "Koskurna",
+ "pos_x": 6.625,
+ "pos_y": 174.6875,
+ "pos_z": -42.75,
+ "stations": "Ziemkiewicz Works,Mitchell Mine,Gaultier de Varennes Vision"
+ },
+ {
+ "name": "Koskurni",
+ "pos_x": 120.6875,
+ "pos_y": -220.1875,
+ "pos_z": 8.78125,
+ "stations": "Verrazzano Installation,Gora Base"
+ },
+ {
+ "name": "Kosraean",
+ "pos_x": -86.71875,
+ "pos_y": -47.21875,
+ "pos_z": 99.96875,
+ "stations": "Hurley Hub,Voss City,Stephenson Colony"
+ },
+ {
+ "name": "Kosretia",
+ "pos_x": 37.3125,
+ "pos_y": -165.40625,
+ "pos_z": 133.0625,
+ "stations": "Mather Terminal,Gorgani Dock,McDaniel Vision,Clark Dock,Kulin Base"
+ },
+ {
+ "name": "Kota Thian",
+ "pos_x": 100.28125,
+ "pos_y": 40.875,
+ "pos_z": 118.75,
+ "stations": "Stevin Terminal,Leichhardt Gateway,Lee Port"
+ },
+ {
+ "name": "Kotait",
+ "pos_x": 86.90625,
+ "pos_y": -24.3125,
+ "pos_z": -72.96875,
+ "stations": "Burke Gateway,Quimper Enterprise,Coulomb Orbital,Cortes Works,Franke Legacy"
+ },
+ {
+ "name": "Kotaition",
+ "pos_x": -100.84375,
+ "pos_y": 35.5,
+ "pos_z": -36.34375,
+ "stations": "Gardner Landing,Bose Settlement"
+ },
+ {
+ "name": "Kotandji",
+ "pos_x": 159.875,
+ "pos_y": -112.96875,
+ "pos_z": 39.03125,
+ "stations": "Henry Station,Sugie Survey,O'Neill Installation"
+ },
+ {
+ "name": "Kotian",
+ "pos_x": -50.75,
+ "pos_y": -127.625,
+ "pos_z": 36.09375,
+ "stations": "Sorayama Station"
+ },
+ {
+ "name": "Kotien",
+ "pos_x": 100.40625,
+ "pos_y": -143.96875,
+ "pos_z": 98.34375,
+ "stations": "Yerka Terminal,Freas Ring,Mallett Horizons,Kirshner Barracks"
+ },
+ {
+ "name": "Kotiga",
+ "pos_x": 131,
+ "pos_y": 23.09375,
+ "pos_z": 68.90625,
+ "stations": "Irwin Mines,Pawelczyk Beacon,Boodt Depot,Burnell's Inheritance"
+ },
+ {
+ "name": "Kotigen",
+ "pos_x": 76.84375,
+ "pos_y": -23.9375,
+ "pos_z": -146.5625,
+ "stations": "Reiter Base,Rasch Installation"
+ },
+ {
+ "name": "Kotigeni",
+ "pos_x": -18.21875,
+ "pos_y": -66.875,
+ "pos_z": -90.0625,
+ "stations": "Taylor Enterprise,Wisniewski-Snerg Keep,Shaw Port"
+ },
+ {
+ "name": "Kotilekui",
+ "pos_x": -82.71875,
+ "pos_y": -51.125,
+ "pos_z": -20.9375,
+ "stations": "Vinge Terminal,Marlowe Dock,Coppel Port,Shaara Stop,Tokarev Beacon,Temple Hub,Altshuller Escape,Abernathy Dock,Lerner Orbital,Cook Dock,Lorrah Port,Darnielle Station,Higginbotham Hub,Hire Survey,Robinson's Folly"
+ },
+ {
+ "name": "Kotilem",
+ "pos_x": 49.1875,
+ "pos_y": -20.1875,
+ "pos_z": 121.90625,
+ "stations": "Gunter Vision,Lunney Port,Pajdusakova Keep"
+ },
+ {
+ "name": "Kotiniez",
+ "pos_x": 94.125,
+ "pos_y": -14.78125,
+ "pos_z": -108.375,
+ "stations": "Bates Platform,Hedin Freeport"
+ },
+ {
+ "name": "Kou Hua",
+ "pos_x": 111.28125,
+ "pos_y": -145.5,
+ "pos_z": 63.25,
+ "stations": "Olahus Hub,Ernst Vision,Carlisle Beacon,Stiles Terminal,Baxter Arsenal"
+ },
+ {
+ "name": "Kou Mantes",
+ "pos_x": 103.96875,
+ "pos_y": -129.625,
+ "pos_z": 83.40625,
+ "stations": "Leberecht Tempel Orbital,Espenak Port,Wingrove Lab,Abetti Survey"
+ },
+ {
+ "name": "Kou Zu",
+ "pos_x": -21.84375,
+ "pos_y": -11.25,
+ "pos_z": -92.65625,
+ "stations": "Edison Orbital,Galilei Landing,Borlaug's Inheritance,Tiedemann Landing,Duffy Settlement"
+ },
+ {
+ "name": "Kovaci",
+ "pos_x": 20.34375,
+ "pos_y": -144.90625,
+ "pos_z": 59.375,
+ "stations": "Veron Survey,Davidson Terminal,Jung Survey,Lee Landing"
+ },
+ {
+ "name": "Kovae",
+ "pos_x": -3.0625,
+ "pos_y": -140.875,
+ "pos_z": 95.5625,
+ "stations": "Fowler Platform"
+ },
+ {
+ "name": "Kovantani",
+ "pos_x": -3.75,
+ "pos_y": 96,
+ "pos_z": 124.78125,
+ "stations": "Nehsi Settlement,Cummings Refinery,Meyrink Port,Navigator Silo,Dias Installation"
+ },
+ {
+ "name": "Kovashco",
+ "pos_x": 151.28125,
+ "pos_y": -70.21875,
+ "pos_z": 25.375,
+ "stations": "Gaensler Outpost,Nakamura Relay"
+ },
+ {
+ "name": "Koya Gu",
+ "pos_x": 134.53125,
+ "pos_y": -225,
+ "pos_z": 68.28125,
+ "stations": "Wigura Vision"
+ },
+ {
+ "name": "Koyang",
+ "pos_x": -120.9375,
+ "pos_y": -78.59375,
+ "pos_z": 48.34375,
+ "stations": "Hand Orbital"
+ },
+ {
+ "name": "Koyans",
+ "pos_x": 140.9375,
+ "pos_y": -142.1875,
+ "pos_z": 58.6875,
+ "stations": "Valz City,Cauchy Terminal,Bainbridge Dock,MacCready Vision,Schaumasse Vision,Bailly Ring,Kuchner Dock,Fremion Depot,Kelly Beacon,Bachman Arsenal"
+ },
+ {
+ "name": "Koyeris",
+ "pos_x": -41.90625,
+ "pos_y": 64.15625,
+ "pos_z": -54.875,
+ "stations": "Fernandes Port,Teng Relay"
+ },
+ {
+ "name": "KP Tauri",
+ "pos_x": -13.46875,
+ "pos_y": -22.53125,
+ "pos_z": -48.6875,
+ "stations": "Spedding Orbital,Kroehl Silo,Anders Orbital,Mohmand Dock,Cavalieri Gateway"
+ },
+ {
+ "name": "Kpaniya",
+ "pos_x": -38.21875,
+ "pos_y": 40.0625,
+ "pos_z": 104,
+ "stations": "Tilman Settlement"
+ },
+ {
+ "name": "Kpannam",
+ "pos_x": -16.8125,
+ "pos_y": -152.96875,
+ "pos_z": 117.625,
+ "stations": "Luu Ring,Hipparchus Dock,Kerr Installation,Frost Terminal"
+ },
+ {
+ "name": "Kpannon Di",
+ "pos_x": -97.25,
+ "pos_y": -70.40625,
+ "pos_z": -95.03125,
+ "stations": "Madsen Base"
+ },
+ {
+ "name": "Kpelentii",
+ "pos_x": 52.5625,
+ "pos_y": -102.34375,
+ "pos_z": 123.8125,
+ "stations": "Bonestell Platform,Bouvard Laboratory"
+ },
+ {
+ "name": "Kpelidoog",
+ "pos_x": 71.3125,
+ "pos_y": -115.21875,
+ "pos_z": -51.96875,
+ "stations": "Hipparchus Terminal,Dufay Vision,Bothezat Hub,Hanke-Woods Hub,Dominique Terminal,Tarter Terminal,Rangarajan Installation,Brothers Dock,Airy Hub,Russo Refinery,Still Krisi's Eclipse,Hickam's Folly"
+ },
+ {
+ "name": "Kpon",
+ "pos_x": 74.25,
+ "pos_y": -224.34375,
+ "pos_z": -38.96875,
+ "stations": "Nahavandi Vision"
+ },
+ {
+ "name": "Kpong",
+ "pos_x": 87.75,
+ "pos_y": -218.15625,
+ "pos_z": 83.25,
+ "stations": "Bell Mine,Trimble Camp"
+ },
+ {
+ "name": "Kponiugo",
+ "pos_x": -81.875,
+ "pos_y": -61.4375,
+ "pos_z": 57.125,
+ "stations": "Wilson Terminal,Lessing Hub"
+ },
+ {
+ "name": "Krangwe",
+ "pos_x": -163.96875,
+ "pos_y": -3.40625,
+ "pos_z": 22.6875,
+ "stations": "Noli Gateway,Ehrenfried Kegel Arsenal,Anders Exchange"
+ },
+ {
+ "name": "Kratakak",
+ "pos_x": 108.375,
+ "pos_y": -72.46875,
+ "pos_z": -77.96875,
+ "stations": "Wingrove Port,Temple Works,Messier Terminal"
+ },
+ {
+ "name": "Krataula",
+ "pos_x": -2.4375,
+ "pos_y": 52.8125,
+ "pos_z": 111.625,
+ "stations": "Watts Vision,Zamyatin Dock,Henderson's Progress"
+ },
+ {
+ "name": "Krataxo",
+ "pos_x": 50.34375,
+ "pos_y": -133.15625,
+ "pos_z": -66.8125,
+ "stations": "Curtis Port,Goodman Colony,Kozin Vision"
+ },
+ {
+ "name": "Krathamani",
+ "pos_x": 11.125,
+ "pos_y": -33.40625,
+ "pos_z": 79.5,
+ "stations": "Hambly Estate,Barjavel Hub,Haber Point"
+ },
+ {
+ "name": "Kraua",
+ "pos_x": 15.75,
+ "pos_y": -122.59375,
+ "pos_z": -118.125,
+ "stations": "Howe Stop"
+ },
+ {
+ "name": "Kraualm",
+ "pos_x": 27.1875,
+ "pos_y": -160.53125,
+ "pos_z": 169.3125,
+ "stations": "Youll Penal colony"
+ },
+ {
+ "name": "Krauanan",
+ "pos_x": 63.9375,
+ "pos_y": 30.90625,
+ "pos_z": 109.3125,
+ "stations": "Hire Camp"
+ },
+ {
+ "name": "Kraudji",
+ "pos_x": 43,
+ "pos_y": -116.625,
+ "pos_z": -95.96875,
+ "stations": "Sakers Terminal"
+ },
+ {
+ "name": "Kraunaha",
+ "pos_x": -142.96875,
+ "pos_y": -20.78125,
+ "pos_z": -70.4375,
+ "stations": "Ochoa Orbital"
+ },
+ {
+ "name": "Kraung",
+ "pos_x": 116.5,
+ "pos_y": -51.34375,
+ "pos_z": -17,
+ "stations": "Wallis Platform"
+ },
+ {
+ "name": "Kraurno",
+ "pos_x": -81.4375,
+ "pos_y": -84.84375,
+ "pos_z": 57.15625,
+ "stations": "Sabine Platform,Morris Installation,Hadamard Base,Gooch Observatory"
+ },
+ {
+ "name": "Kraz",
+ "pos_x": 99.1875,
+ "pos_y": 91.8125,
+ "pos_z": 54.25,
+ "stations": "Lind Refinery"
+ },
+ {
+ "name": "Kremainn",
+ "pos_x": -37.0625,
+ "pos_y": -11.15625,
+ "pos_z": 59.0625,
+ "stations": "Wohler Terminal,Volta Station,Viete Beacon,Curbeam Hub"
+ },
+ {
+ "name": "Kremata",
+ "pos_x": 113.9375,
+ "pos_y": -2.34375,
+ "pos_z": -54.625,
+ "stations": "Gardner Horizons,Fullerton Ring"
+ },
+ {
+ "name": "Kremavi",
+ "pos_x": -136.875,
+ "pos_y": -74.125,
+ "pos_z": 25.6875,
+ "stations": "White Station,Spedding Base,Kratman Relay,Tem's Inheritance"
+ },
+ {
+ "name": "Kremovi",
+ "pos_x": 144.46875,
+ "pos_y": -154.40625,
+ "pos_z": 30.90625,
+ "stations": "Kawasato Orbital,Merrill Barracks,Trimble Terminal,Otomo Terminal,Cottenot Hub,Dawes Orbital,Coye Holdings,Leydenfrost Vision,Kibalchich Orbital,Mouchez Orbital,Oort Vision,Brothers Settlement"
+ },
+ {
+ "name": "Krinayaka",
+ "pos_x": 147.78125,
+ "pos_y": -174.6875,
+ "pos_z": 82.6875,
+ "stations": "Darwin Prospect"
+ },
+ {
+ "name": "Krinbea",
+ "pos_x": 111.28125,
+ "pos_y": -80.125,
+ "pos_z": -83.53125,
+ "stations": "Albategnius Port,Hanke-Woods Dock,Meinel Dock,Ikeya Vision,Emshwiller City,Elst's Progress"
+ },
+ {
+ "name": "Krinda",
+ "pos_x": -150.9375,
+ "pos_y": -0.84375,
+ "pos_z": -51.1875,
+ "stations": "Xiaoguan Station,King Holdings"
+ },
+ {
+ "name": "Krine",
+ "pos_x": -6.46875,
+ "pos_y": -173.5625,
+ "pos_z": -37.09375,
+ "stations": "Shaw Port"
+ },
+ {
+ "name": "Krinjmarini",
+ "pos_x": 156.375,
+ "pos_y": -58.59375,
+ "pos_z": -96.8125,
+ "stations": "Makeev Works,Cayley Vision"
+ },
+ {
+ "name": "Krinu",
+ "pos_x": 106.4375,
+ "pos_y": -216.46875,
+ "pos_z": 103.96875,
+ "stations": "Neumann Works"
+ },
+ {
+ "name": "Krisha",
+ "pos_x": -108.625,
+ "pos_y": -107.21875,
+ "pos_z": 14.65625,
+ "stations": "Fincke Depot,Garan Survey"
+ },
+ {
+ "name": "Krist",
+ "pos_x": 74,
+ "pos_y": 15.6875,
+ "pos_z": -95.625,
+ "stations": "Dann Terminal,Searfoss Reach,Wilson Exchange,Foden City,Herreshoff Vision"
+ },
+ {
+ "name": "Kriva",
+ "pos_x": 57.84375,
+ "pos_y": 138.8125,
+ "pos_z": 73.03125,
+ "stations": "Popov Prospect,Bartlett Penal colony,Coulter Installation"
+ },
+ {
+ "name": "Krivasho",
+ "pos_x": -101.375,
+ "pos_y": -84,
+ "pos_z": -76.9375,
+ "stations": "Morgan Dock,Asimov Horizons,Potrykus Platform"
+ },
+ {
+ "name": "Krivi",
+ "pos_x": -67.625,
+ "pos_y": 101.8125,
+ "pos_z": 54.28125,
+ "stations": "Higginbotham Hub,Ansari Gateway,Aristotle Colony,Tudela Survey,Huberath Silo"
+ },
+ {
+ "name": "Krivia",
+ "pos_x": -36.84375,
+ "pos_y": -129.40625,
+ "pos_z": 108,
+ "stations": "Langford Terminal,Hyecho City,Salgari Dock"
+ },
+ {
+ "name": "Krivisci",
+ "pos_x": -84.84375,
+ "pos_y": -79.1875,
+ "pos_z": 120.3125,
+ "stations": "Curbeam Dock,Vasyutin Plant"
+ },
+ {
+ "name": "Krobrigae",
+ "pos_x": 64.84375,
+ "pos_y": -80.4375,
+ "pos_z": -18.375,
+ "stations": "Shunkai Horizons"
+ },
+ {
+ "name": "Krobrigurra",
+ "pos_x": 140.28125,
+ "pos_y": -89,
+ "pos_z": 71.5625,
+ "stations": "Duque Port,Velho Horizons,Searle Dock,Brashear Base"
+ },
+ {
+ "name": "Kruger 60",
+ "pos_x": -12.625,
+ "pos_y": 0,
+ "pos_z": -3.40625,
+ "stations": "Kepler Gateway,O'Brien Depot"
+ },
+ {
+ "name": "Krumandaqu",
+ "pos_x": 102.6875,
+ "pos_y": -131.40625,
+ "pos_z": 35.90625,
+ "stations": "Ayers Reach,Barbuy Relay"
+ },
+ {
+ "name": "Krumine",
+ "pos_x": -154.90625,
+ "pos_y": 17.53125,
+ "pos_z": -79.03125,
+ "stations": "Clifford's Progress,Barth's Inheritance,Lynn Escape"
+ },
+ {
+ "name": "Kuan Gu",
+ "pos_x": 48.28125,
+ "pos_y": -111.875,
+ "pos_z": -50.25,
+ "stations": "McMillan Terminal"
+ },
+ {
+ "name": "Kuan Ti",
+ "pos_x": 75.75,
+ "pos_y": -81.15625,
+ "pos_z": 38.25,
+ "stations": "Niemeyer Hub,Truly Terminal,McCool Landing"
+ },
+ {
+ "name": "Kuan Yin",
+ "pos_x": -20.53125,
+ "pos_y": 61.25,
+ "pos_z": -140.96875,
+ "stations": "Gibson Penal colony,Ramsbottom Enterprise,Morgan Port,Wescott Station,Ehrenfried Kegel Terminal,Luiken's Folly"
+ },
+ {
+ "name": "Kuanes",
+ "pos_x": 87.90625,
+ "pos_y": -0.53125,
+ "pos_z": -32.28125,
+ "stations": "Hubble Hub,Torricelli Hub,Spring Vision,Altshuller Installation,Haber's Inheritance"
+ },
+ {
+ "name": "Kuang",
+ "pos_x": 55.0625,
+ "pos_y": -93.625,
+ "pos_z": 90.5,
+ "stations": "Frazetta Platform,Korolev Mines,Secchi Survey"
+ },
+ {
+ "name": "Kuanga",
+ "pos_x": 3.71875,
+ "pos_y": -71.5,
+ "pos_z": -65.03125,
+ "stations": "Poincare Oasis,Harrison Refinery,Helms City"
+ },
+ {
+ "name": "Kuangwutja",
+ "pos_x": 74.4375,
+ "pos_y": -33.65625,
+ "pos_z": 82.125,
+ "stations": "Oshima Landing,Dishoeck Vision"
+ },
+ {
+ "name": "Kucub Hua",
+ "pos_x": -10.65625,
+ "pos_y": 73.28125,
+ "pos_z": 65.625,
+ "stations": "Helmholtz Installation,Cenker Landing"
+ },
+ {
+ "name": "Kucumana",
+ "pos_x": -81.25,
+ "pos_y": 38.28125,
+ "pos_z": 26.125,
+ "stations": "Thorne Port,Cheli's Progress,Lyell Dock"
+ },
+ {
+ "name": "Kudi",
+ "pos_x": -64.25,
+ "pos_y": 95.53125,
+ "pos_z": -74.6875,
+ "stations": "Silves Horizons,Morey Silo"
+ },
+ {
+ "name": "Kudiyu",
+ "pos_x": -74.53125,
+ "pos_y": -46.6875,
+ "pos_z": -74.71875,
+ "stations": "Khan Holdings,Sharman Arsenal,Gurragchaa Colony"
+ },
+ {
+ "name": "Kugaitu",
+ "pos_x": 71.90625,
+ "pos_y": -40.625,
+ "pos_z": -121.21875,
+ "stations": "Hovell Dock"
+ },
+ {
+ "name": "Kugay",
+ "pos_x": 31.59375,
+ "pos_y": -94.625,
+ "pos_z": -72.09375,
+ "stations": "Shepherd Dock,Grant Dock"
+ },
+ {
+ "name": "Kugaybaks",
+ "pos_x": -23.84375,
+ "pos_y": 1.375,
+ "pos_z": -141.8125,
+ "stations": "Barnes Survey,Lenthall Beacon"
+ },
+ {
+ "name": "KUI 11",
+ "pos_x": -52.5,
+ "pos_y": 4.375,
+ "pos_z": -58.9375,
+ "stations": "Bagian Terminal,Gohar City,Penn Prospect,Piccard Point,Hopkins Ring,Behnken Port,Foreman Orbital,Jenner Hub,Shaikh's Progress,Guest Gateway,Tennyson d'Eyncourt's Progress"
+ },
+ {
+ "name": "Kui Hsien",
+ "pos_x": 45.15625,
+ "pos_y": -101.46875,
+ "pos_z": 11.875,
+ "stations": "Stuart Mine,Saavedra Dock"
+ },
+ {
+ "name": "Kui Xenab",
+ "pos_x": 43.75,
+ "pos_y": -100.5625,
+ "pos_z": -23.34375,
+ "stations": "Lemaitre Depot,Paczynski Hub,d'Allonville City"
+ },
+ {
+ "name": "Kui Xiani",
+ "pos_x": 40.6875,
+ "pos_y": 13.125,
+ "pos_z": 102.78125,
+ "stations": "Bulychev Ring,Kennicott Depot,Offutt Horizons"
+ },
+ {
+ "name": "Kui Xiba",
+ "pos_x": -4.25,
+ "pos_y": 92.03125,
+ "pos_z": -43.34375,
+ "stations": "Kube-McDowell Depot,Smith Works,Fernandes de Queiros Survey"
+ },
+ {
+ "name": "Kui Xing",
+ "pos_x": -90.78125,
+ "pos_y": -49.21875,
+ "pos_z": 21.625,
+ "stations": "Andreas City,Henricks Dock,Thornycroft Arsenal"
+ },
+ {
+ "name": "Kuja",
+ "pos_x": -45.78125,
+ "pos_y": 67,
+ "pos_z": 111,
+ "stations": "Snyder Stop"
+ },
+ {
+ "name": "Kuk",
+ "pos_x": -21.28125,
+ "pos_y": 69.09375,
+ "pos_z": -16.3125,
+ "stations": "Prospector's Rest,Veblen City,Evans Station,Perga City,Eudoxus Dock,Harper Horizons,Napier Platform,Harrison Enterprise"
+ },
+ {
+ "name": "Kukas",
+ "pos_x": 131.78125,
+ "pos_y": -20.375,
+ "pos_z": 77,
+ "stations": "Laing's Folly,Oberth Point"
+ },
+ {
+ "name": "Kuki An",
+ "pos_x": 151.84375,
+ "pos_y": -155.75,
+ "pos_z": 84.9375,
+ "stations": "Paola Prospect,Henson Gateway,Weill Landing,Ryle Prospect,Bokeili Hub"
+ },
+ {
+ "name": "Kukuan",
+ "pos_x": 95.40625,
+ "pos_y": -48.59375,
+ "pos_z": 57.40625,
+ "stations": "Olivas Camp,Babbage Point"
+ },
+ {
+ "name": "Kukula",
+ "pos_x": -65.34375,
+ "pos_y": -87.84375,
+ "pos_z": 122.46875,
+ "stations": "Henricks Platform,Binder Vision,Tesla Platform"
+ },
+ {
+ "name": "Kulaye",
+ "pos_x": 95.53125,
+ "pos_y": -93.6875,
+ "pos_z": 61.125,
+ "stations": "Vyssotsky Installation"
+ },
+ {
+ "name": "Kulianminy",
+ "pos_x": -5.03125,
+ "pos_y": -153.5,
+ "pos_z": -33.34375,
+ "stations": "Brashear Terminal,Darwin Hub,Zarnecki Plant,Wilson Prospect"
+ },
+ {
+ "name": "Kulici",
+ "pos_x": 18.125,
+ "pos_y": -90.09375,
+ "pos_z": -73.21875,
+ "stations": "Araki Vision,Thornycroft Settlement,Angstrom Hub,Vance Survey,Mayer Hub"
+ },
+ {
+ "name": "Kuligans",
+ "pos_x": -23.46875,
+ "pos_y": 83.375,
+ "pos_z": -58.84375,
+ "stations": "Adams Port,Russell Terminal,Bohnhoff Gateway,Muhammad Ibn Battuta City,Flynn Hub,Tiedemann Station,Tucker Ring,Dedman Terminal,Monge Station,Marusek City,Shunn Vista"
+ },
+ {
+ "name": "Kulillechet",
+ "pos_x": 153.75,
+ "pos_y": 39.90625,
+ "pos_z": 67.03125,
+ "stations": "Fontenay Settlement,Anderson Terminal,Soper Vista,de Caminha Mines,Bulychev's Claim"
+ },
+ {
+ "name": "Kulingga",
+ "pos_x": -70.96875,
+ "pos_y": 33.8125,
+ "pos_z": -73.84375,
+ "stations": "Vogel Gateway,Alvarado Bastion,Virtanen Lab,Kendrick Colony,Bennett Hub"
+ },
+ {
+ "name": "Kulinniya",
+ "pos_x": 32.5,
+ "pos_y": 71.96875,
+ "pos_z": -142.75,
+ "stations": "Wylie Base,Shatalov Hub"
+ },
+ {
+ "name": "Kulis",
+ "pos_x": -1.0625,
+ "pos_y": -48.3125,
+ "pos_z": 138.34375,
+ "stations": "Shepard Station,Hurston Holdings"
+ },
+ {
+ "name": "Kulispeling",
+ "pos_x": 138.6875,
+ "pos_y": 38.8125,
+ "pos_z": 19.5625,
+ "stations": "Wallis' Folly,Clute Mines"
+ },
+ {
+ "name": "Kulkan Lung",
+ "pos_x": 67.71875,
+ "pos_y": -10.5,
+ "pos_z": 98.78125,
+ "stations": "Gell-Mann Enterprise,Brunner Arena,Yamazaki City,Walters Depot,Searfoss Dock,Celsius Dock,Herndon Base"
+ },
+ {
+ "name": "Kultultuls",
+ "pos_x": 165.40625,
+ "pos_y": -161.8125,
+ "pos_z": -19.625,
+ "stations": "Allen St. John Base,Gerlache Bastion"
+ },
+ {
+ "name": "Kulungan",
+ "pos_x": -77.09375,
+ "pos_y": 58.59375,
+ "pos_z": -52.9375,
+ "stations": "Bursch Enterprise,Napier Station,von Helmont Enterprise,Pierce Hub,Dirichlet Survey"
+ },
+ {
+ "name": "Kuluujja",
+ "pos_x": -80.4375,
+ "pos_y": 19.0625,
+ "pos_z": 99.8125,
+ "stations": "Olsen Plant,Kelly Settlement,Simonyi Orbital,Rontgen City,la Cosa Beacon"
+ },
+ {
+ "name": "Kuma",
+ "pos_x": -82.15625,
+ "pos_y": 53.71875,
+ "pos_z": 10.0625,
+ "stations": "Elion Dock,Divis Port,Dobrovolskiy Orbital,Duffy Orbital,Brandenstein Port,Clerk Prospect,Stith Arsenal,Godwin Landing"
+ },
+ {
+ "name": "Kumal",
+ "pos_x": 161.03125,
+ "pos_y": -188.15625,
+ "pos_z": 100.0625,
+ "stations": "Lilienthal Works"
+ },
+ {
+ "name": "Kumana",
+ "pos_x": 72.28125,
+ "pos_y": 30.0625,
+ "pos_z": -22.5625,
+ "stations": "Rosenberg Dock,Hopkinson Refinery,Ellis Settlement"
+ },
+ {
+ "name": "Kumata",
+ "pos_x": -150.96875,
+ "pos_y": 67.03125,
+ "pos_z": -37.8125,
+ "stations": "Vishweswarayya Enterprise,al-Khayyam Hub,Detmer Hub,Fisher Station,Tiedemann Prospect"
+ },
+ {
+ "name": "Kumbardin",
+ "pos_x": 20.78125,
+ "pos_y": -232.875,
+ "pos_z": 68.59375,
+ "stations": "Maanen Beacon"
+ },
+ {
+ "name": "Kumbaya",
+ "pos_x": 64.125,
+ "pos_y": 91.28125,
+ "pos_z": 164.09375,
+ "stations": "Heceta Landing,Zamyatin Mine,Tilley Works"
+ },
+ {
+ "name": "Kumbia",
+ "pos_x": -44.5625,
+ "pos_y": -28.90625,
+ "pos_z": 154.40625,
+ "stations": "Hope Hub"
+ },
+ {
+ "name": "Kumbou",
+ "pos_x": 74.40625,
+ "pos_y": -201.03125,
+ "pos_z": -28.5,
+ "stations": "Kosai Landing,Dilworth Laboratory,Thome Vision"
+ },
+ {
+ "name": "Kumbwe",
+ "pos_x": 121,
+ "pos_y": -7.3125,
+ "pos_z": -14.8125,
+ "stations": "Samokutyayev Prospect"
+ },
+ {
+ "name": "Kume",
+ "pos_x": -148.5625,
+ "pos_y": 43.46875,
+ "pos_z": -37.3125,
+ "stations": "Narvaez Plant,Stasheff Outpost"
+ },
+ {
+ "name": "Kumod",
+ "pos_x": 105.9375,
+ "pos_y": -157.0625,
+ "pos_z": 89.0625,
+ "stations": "van Royen Port,Harawi Platform"
+ },
+ {
+ "name": "Kumokum",
+ "pos_x": 76.3125,
+ "pos_y": 16.78125,
+ "pos_z": 103.875,
+ "stations": "Perry Relay,Oliver Refinery"
+ },
+ {
+ "name": "Kumui",
+ "pos_x": 117.15625,
+ "pos_y": -66.0625,
+ "pos_z": -41.65625,
+ "stations": "Naboth Hangar,Kimura Settlement,Heinkel Installation"
+ },
+ {
+ "name": "Kun",
+ "pos_x": -134.65625,
+ "pos_y": -46.875,
+ "pos_z": 45.375,
+ "stations": "Bresnik Colony"
+ },
+ {
+ "name": "Kunab",
+ "pos_x": -98.53125,
+ "pos_y": -20.71875,
+ "pos_z": -62.46875,
+ "stations": "Rorschach Hangar,Maudslay Station,McDivitt Installation"
+ },
+ {
+ "name": "Kunabi",
+ "pos_x": 47.1875,
+ "pos_y": 46.9375,
+ "pos_z": -81.125,
+ "stations": "Roberts Hangar,Greenleaf Arsenal"
+ },
+ {
+ "name": "Kunah",
+ "pos_x": -7.28125,
+ "pos_y": -134.90625,
+ "pos_z": 113.125,
+ "stations": "Hiyya Beacon,Charlois Stop"
+ },
+ {
+ "name": "Kunaheo",
+ "pos_x": 101.625,
+ "pos_y": 1.8125,
+ "pos_z": 115.4375,
+ "stations": "Russell Platform,al-Haytham Dock,Birkhoff Prospect,Hannu Base"
+ },
+ {
+ "name": "Kunahpu",
+ "pos_x": 78.46875,
+ "pos_y": 7.15625,
+ "pos_z": -40.25,
+ "stations": "Evangelisti Base,Edgeworth Plant,Noguchi Prospect,Parker Holdings"
+ },
+ {
+ "name": "Kunapa",
+ "pos_x": 172.875,
+ "pos_y": -218.8125,
+ "pos_z": -8.625,
+ "stations": "Gurevich Port"
+ },
+ {
+ "name": "Kunbudj",
+ "pos_x": 148.84375,
+ "pos_y": 34.25,
+ "pos_z": -21.03125,
+ "stations": "Lasswitz Base,Stein Gateway,Kovalevskaya Installation"
+ },
+ {
+ "name": "Kunbuluan",
+ "pos_x": -90.09375,
+ "pos_y": 61.6875,
+ "pos_z": -30.875,
+ "stations": "Drummond Camp,Swigert Port"
+ },
+ {
+ "name": "Kunbure",
+ "pos_x": 66.8125,
+ "pos_y": -222.03125,
+ "pos_z": 119.75,
+ "stations": "Truly Installation,Herzog Terminal,Molyneux Prospect,Tasman Relay"
+ },
+ {
+ "name": "Kunda",
+ "pos_x": -7,
+ "pos_y": -193.53125,
+ "pos_z": 84.46875,
+ "stations": "Pickering Arsenal,Wright Mines"
+ },
+ {
+ "name": "Kundalini",
+ "pos_x": 161.53125,
+ "pos_y": -161.59375,
+ "pos_z": -56.4375,
+ "stations": "Greenstein Beacon"
+ },
+ {
+ "name": "Kundinke",
+ "pos_x": 187.8125,
+ "pos_y": -120.28125,
+ "pos_z": 81.5,
+ "stations": "Sandage's Inheritance,Ozanne Penal colony"
+ },
+ {
+ "name": "Kundjabozo",
+ "pos_x": -63.53125,
+ "pos_y": -115.15625,
+ "pos_z": 16.875,
+ "stations": "Carlisle Penal colony,Phillips Platform"
+ },
+ {
+ "name": "Kundjiban",
+ "pos_x": 159.125,
+ "pos_y": -99.96875,
+ "pos_z": 89.09375,
+ "stations": "Kirby Orbital,van de Hulst Enterprise,Dyson Base,Honda Laboratory"
+ },
+ {
+ "name": "Kung Mu",
+ "pos_x": -92.8125,
+ "pos_y": -16.21875,
+ "pos_z": -35.65625,
+ "stations": "Wohler Station,Lazutkin Port,Seddon Orbital,Morey Orbital,Harvey City,Moore Point,Hawley City,Tyson Town,Foda Port,Zoline Hub"
+ },
+ {
+ "name": "Kung Te",
+ "pos_x": -93.40625,
+ "pos_y": -37.71875,
+ "pos_z": -2.875,
+ "stations": "Krylov Hub,Dickson Gateway,Vonarburg City,Bellamy Enterprise,Conway Observatory,Scithers' Inheritance,Houtman Terminal,Timofeyevich Orbital,Thomas Settlement,MacKellar Enterprise,Bounds Gateway,Sheckley Hub"
+ },
+ {
+ "name": "Kunga",
+ "pos_x": -169.90625,
+ "pos_y": -23.375,
+ "pos_z": 37.5,
+ "stations": "Mach Station,Bushnell Gateway,Seega Colony,Pratchett Arsenal,Popov Relay"
+ },
+ {
+ "name": "Kungadutji",
+ "pos_x": 109.59375,
+ "pos_y": 15.21875,
+ "pos_z": 95.0625,
+ "stations": "Marusek City,Rose Station,Petaja Enterprise,McAuley Vision,Veblen Point,Underwood Holdings"
+ },
+ {
+ "name": "Kungantju",
+ "pos_x": 62.25,
+ "pos_y": -51.96875,
+ "pos_z": -60.28125,
+ "stations": "Copernicus City,von Helmont City"
+ },
+ {
+ "name": "Kungati",
+ "pos_x": -123.5,
+ "pos_y": 13.25,
+ "pos_z": -35.78125,
+ "stations": "Elvstrom Prospect,Bernoulli Terminal"
+ },
+ {
+ "name": "Kunggalerni",
+ "pos_x": 31.78125,
+ "pos_y": -180,
+ "pos_z": 26.96875,
+ "stations": "Bus Vision,Sheepshanks Dock,Wilhelm von Struve Settlement,Bering Observatory"
+ },
+ {
+ "name": "Kunggari",
+ "pos_x": -69.96875,
+ "pos_y": -78.09375,
+ "pos_z": 141.21875,
+ "stations": "Balmer Ring,Bernoulli Enterprise,Menezes Hub,Wylie Terminal,Rice Depot"
+ },
+ {
+ "name": "Kunggi",
+ "pos_x": 38.71875,
+ "pos_y": 6.0625,
+ "pos_z": 29.25,
+ "stations": "Vesalius City,Atkov Hub,Marshburn Gateway,Rashid Port"
+ },
+ {
+ "name": "Kunggua",
+ "pos_x": 83.03125,
+ "pos_y": -42.90625,
+ "pos_z": 83.25,
+ "stations": "Szilard Gateway,Bouch Gateway"
+ },
+ {
+ "name": "Kunggul",
+ "pos_x": 47.40625,
+ "pos_y": -25.34375,
+ "pos_z": 102.65625,
+ "stations": "Northrop Gateway"
+ },
+ {
+ "name": "Kungkalenja",
+ "pos_x": 61.28125,
+ "pos_y": 115.875,
+ "pos_z": -0.5625,
+ "stations": "Zholobov Hub,Runco Ring,Bates Holdings,Piaget Hub,Klink Bastion"
+ },
+ {
+ "name": "Kungkalis",
+ "pos_x": 54.28125,
+ "pos_y": -109.5625,
+ "pos_z": 138.21875,
+ "stations": "Struve Hangar,Gunn Arena,Laird Keep"
+ },
+ {
+ "name": "Kungo",
+ "pos_x": -23.40625,
+ "pos_y": 104.25,
+ "pos_z": -67.25,
+ "stations": "Beaumont Market,Vaez de Torres' Progress,Merle Observatory,Baker Barracks,Geston Point"
+ },
+ {
+ "name": "Kungulmas",
+ "pos_x": -99.0625,
+ "pos_y": 55.03125,
+ "pos_z": 59.03125,
+ "stations": "Gotlieb Gateway,Atiyah Reach"
+ },
+ {
+ "name": "Kungun",
+ "pos_x": 68.75,
+ "pos_y": -66.5,
+ "pos_z": 21.46875,
+ "stations": "Hewish Colony,Lowell Hangar,Lee Beacon"
+ },
+ {
+ "name": "Kungurutii",
+ "pos_x": 17.84375,
+ "pos_y": -17.5625,
+ "pos_z": -71.5625,
+ "stations": "Jett Dock,Lawrence Colony"
+ },
+ {
+ "name": "Kunie",
+ "pos_x": 33.1875,
+ "pos_y": -102.71875,
+ "pos_z": 91.03125,
+ "stations": "Finlay-Freundlich Colony,Shimizu Orbital,Fidalgo Works"
+ },
+ {
+ "name": "Kunj Hef",
+ "pos_x": -12.90625,
+ "pos_y": 20.125,
+ "pos_z": 117,
+ "stations": "Bain Settlement"
+ },
+ {
+ "name": "Kunjak",
+ "pos_x": 42.5,
+ "pos_y": 43.0625,
+ "pos_z": -103.375,
+ "stations": "McQuay Orbital,Muhammad Ibn Battuta Hangar,Hugh Refinery"
+ },
+ {
+ "name": "Kunta",
+ "pos_x": 43.09375,
+ "pos_y": 87.25,
+ "pos_z": -67.21875,
+ "stations": "Szentmartony's Progress,Brash Dock,Lucretius Platform"
+ },
+ {
+ "name": "Kuntae",
+ "pos_x": -61.4375,
+ "pos_y": 93.09375,
+ "pos_z": -50.15625,
+ "stations": "Tognini Horizons,Weber City,Heinlein Works,Brooks Depot,Scully-Power's Progress"
+ },
+ {
+ "name": "Kunth",
+ "pos_x": 65.84375,
+ "pos_y": 88.71875,
+ "pos_z": 38.71875,
+ "stations": "Clement Colony"
+ },
+ {
+ "name": "Kunti",
+ "pos_x": 88.65625,
+ "pos_y": -59.625,
+ "pos_z": -4.0625,
+ "stations": "Hughes Enterprise,Syromyatnikov Terminal"
+ },
+ {
+ "name": "Kuntitak",
+ "pos_x": -64.125,
+ "pos_y": 86.8125,
+ "pos_z": 78.28125,
+ "stations": "Finch's Pride,Fidalgo Dock"
+ },
+ {
+ "name": "Kunuvii",
+ "pos_x": -11.4375,
+ "pos_y": 18.03125,
+ "pos_z": -107.0625,
+ "stations": "Weil Station,Baraniecki Base,Maire Relay,Rescue Ship - Weil Station"
+ },
+ {
+ "name": "Kuo Ti",
+ "pos_x": -13.75,
+ "pos_y": -92.71875,
+ "pos_z": 147.375,
+ "stations": "Feoktistov Station,Curbeam Hub"
+ },
+ {
+ "name": "Kuo Zhi",
+ "pos_x": 27.96875,
+ "pos_y": 76.8125,
+ "pos_z": 62.5625,
+ "stations": "Nachtigal Landing,Swainson Dock,Ising Prospect"
+ },
+ {
+ "name": "Kupala",
+ "pos_x": 39.90625,
+ "pos_y": 97.28125,
+ "pos_z": 62.53125,
+ "stations": "Ostwald Outpost,Blalock Terminal"
+ },
+ {
+ "name": "Kupalites",
+ "pos_x": -140.3125,
+ "pos_y": -8.71875,
+ "pos_z": -11.625,
+ "stations": "Silverberg Hub,Hawkes Depot"
+ },
+ {
+ "name": "Kupalon Hua",
+ "pos_x": 29.25,
+ "pos_y": -204.125,
+ "pos_z": 67.0625,
+ "stations": "Juan de la Cierva Mines,Stiles Base,Sleator Relay"
+ },
+ {
+ "name": "Kupan",
+ "pos_x": 1.09375,
+ "pos_y": -101.3125,
+ "pos_z": 70.125,
+ "stations": "Penrose Orbital,Burnham Orbital,Schwarzschild Port,Taylor Refinery"
+ },
+ {
+ "name": "Kupana",
+ "pos_x": -20.625,
+ "pos_y": 81.28125,
+ "pos_z": -142.28125,
+ "stations": "Hume Port,Conklin Hub,Stafford's Folly"
+ },
+ {
+ "name": "Kuparilda",
+ "pos_x": 147.96875,
+ "pos_y": -118.28125,
+ "pos_z": 10.4375,
+ "stations": "O'Neill Port,Oshima Hub,Antoniadi Penal colony"
+ },
+ {
+ "name": "Kupol Bumba",
+ "pos_x": 51.03125,
+ "pos_y": -12.78125,
+ "pos_z": 79.84375,
+ "stations": "Rennie Hub"
+ },
+ {
+ "name": "Kupol Vuh",
+ "pos_x": -69.4375,
+ "pos_y": -179.625,
+ "pos_z": 4.375,
+ "stations": "May Gateway,Kingsbury Hub,Quick Observatory,Naddoddur Keep"
+ },
+ {
+ "name": "Kupole",
+ "pos_x": -5.875,
+ "pos_y": -136.90625,
+ "pos_z": 90.15625,
+ "stations": "De Camp"
+ },
+ {
+ "name": "Kupoledari",
+ "pos_x": 131.625,
+ "pos_y": 78.03125,
+ "pos_z": 22.96875,
+ "stations": "Wiberg Dock,Padalka Outpost,Kozlov Prospect,Fiennes Plant"
+ },
+ {
+ "name": "Kupoli",
+ "pos_x": 66.78125,
+ "pos_y": -55.75,
+ "pos_z": -70.59375,
+ "stations": "Xing Platform"
+ },
+ {
+ "name": "Kured",
+ "pos_x": 15.625,
+ "pos_y": -95.40625,
+ "pos_z": 11.96875,
+ "stations": "Kuchner Vision,Vogel Port,Tange Terminal,Siegel Depot"
+ },
+ {
+ "name": "Kureinji",
+ "pos_x": 29.78125,
+ "pos_y": -178.78125,
+ "pos_z": -42.59375,
+ "stations": "Weill's Pride"
+ },
+ {
+ "name": "Kureserians",
+ "pos_x": 159.5,
+ "pos_y": -200.875,
+ "pos_z": 78.53125,
+ "stations": "Tepper Installation,Hevelius' Inheritance,Lambert Vision,Dickinson Vision,Finlay Survey,Hale Prospect"
+ },
+ {
+ "name": "Kuri Hsing",
+ "pos_x": -98.71875,
+ "pos_y": -1.53125,
+ "pos_z": -70.78125,
+ "stations": "North Terminal,Quimper Orbital,Goldstein Enterprise,Novitski Arsenal,Clapperton Prospect"
+ },
+ {
+ "name": "Kurians",
+ "pos_x": 125.71875,
+ "pos_y": -101.21875,
+ "pos_z": -8.46875,
+ "stations": "Fabricius Dock"
+ },
+ {
+ "name": "Kurichchan",
+ "pos_x": -100.21875,
+ "pos_y": -47.46875,
+ "pos_z": 37.5,
+ "stations": "Levinson Gateway,Russo Beacon,Brunner Oasis"
+ },
+ {
+ "name": "Kurichime",
+ "pos_x": 150.15625,
+ "pos_y": -176.9375,
+ "pos_z": 49.5,
+ "stations": "Shirazi Mines,Dyson Base,Parker Lab,Wegner Landing"
+ },
+ {
+ "name": "Kuridwen",
+ "pos_x": 147.78125,
+ "pos_y": 37.125,
+ "pos_z": 11.25,
+ "stations": "Dzhanibekov Gateway,Williams Hub,Shalatula Settlement"
+ },
+ {
+ "name": "Kurigosages",
+ "pos_x": 60,
+ "pos_y": 22.15625,
+ "pos_z": 137.125,
+ "stations": "Moore Vision,Rond d'Alembert Colony,Abasheli Barracks,RenenBellot Base"
+ },
+ {
+ "name": "Kurine",
+ "pos_x": 77.9375,
+ "pos_y": -252.40625,
+ "pos_z": -28.46875,
+ "stations": "Carsono Mine,Elmore's Progress,Vaisala Dock"
+ },
+ {
+ "name": "Kuring",
+ "pos_x": -6.9375,
+ "pos_y": 19.4375,
+ "pos_z": 105.5625,
+ "stations": "Vries Installation"
+ },
+ {
+ "name": "Kuringgai",
+ "pos_x": -108.9375,
+ "pos_y": -123.5625,
+ "pos_z": -20.21875,
+ "stations": "Payne Horizons,Budrys Colony,Compton Vision,d'Eyncourt Base"
+ },
+ {
+ "name": "Kurite",
+ "pos_x": 72.71875,
+ "pos_y": -208.65625,
+ "pos_z": -44.8125,
+ "stations": "Sorayama Relay"
+ },
+ {
+ "name": "Kurma",
+ "pos_x": 136.46875,
+ "pos_y": -60.28125,
+ "pos_z": 77.59375,
+ "stations": "Chun Horizons,Luu City,Nadiradze Plant"
+ },
+ {
+ "name": "Kurmodiae",
+ "pos_x": -30.46875,
+ "pos_y": 23,
+ "pos_z": 72.46875,
+ "stations": "Covey Terminal,Virchow Dock,Anderson Orbital,Jones Terminal,Czerny Enterprise,Jernigan Dock,Malyshev Terminal,Surayev Station"
+ },
+ {
+ "name": "Kurna",
+ "pos_x": 149.78125,
+ "pos_y": -103.75,
+ "pos_z": 41.375,
+ "stations": "Hampson's Claim,Phillips Installation"
+ },
+ {
+ "name": "Kurnai",
+ "pos_x": 68.75,
+ "pos_y": 126,
+ "pos_z": 99.78125,
+ "stations": "Banks Station,Svavarsson Ring,White Vision,Stableford Market"
+ },
+ {
+ "name": "Kurra",
+ "pos_x": 46.03125,
+ "pos_y": 65.84375,
+ "pos_z": 116.53125,
+ "stations": "Jones Station"
+ },
+ {
+ "name": "Kurrae",
+ "pos_x": 32.28125,
+ "pos_y": -74.1875,
+ "pos_z": 83.1875,
+ "stations": "Camm Market,Carpenter City,Dawson Ring,Lewis Lab,Houtman Prospect,Denton Horizons,Liouville Relay,Ocampo Point"
+ },
+ {
+ "name": "Kurrama",
+ "pos_x": -5.34375,
+ "pos_y": 82.6875,
+ "pos_z": 68.46875,
+ "stations": "Bertin Hub,Phillips Orbital,Gurney Observatory"
+ },
+ {
+ "name": "Kurrigurr",
+ "pos_x": 57.59375,
+ "pos_y": 32.8125,
+ "pos_z": -99.1875,
+ "stations": "Alexandria Station,Gidzenko Port,Jenner Port,Ross Port,Novitski Terminal,White Barracks,Dutton Holdings"
+ },
+ {
+ "name": "Kuru",
+ "pos_x": -18.53125,
+ "pos_y": 100,
+ "pos_z": 82.09375,
+ "stations": "Carstensz Dock,Weiss Gateway,Antonio de Andrade Survey,Salak Terminal,Perez Town"
+ },
+ {
+ "name": "Kuruacani",
+ "pos_x": -37.71875,
+ "pos_y": -60.53125,
+ "pos_z": 71.28125,
+ "stations": "Moore Enterprise,den Berg Dock,Wheeler Port,Fujimori Hub"
+ },
+ {
+ "name": "Kuruages",
+ "pos_x": -38.59375,
+ "pos_y": 41.75,
+ "pos_z": 60.21875,
+ "stations": "Hernandez Market,Sharp Base"
+ },
+ {
+ "name": "Kurughnaye",
+ "pos_x": 44.71875,
+ "pos_y": -56.46875,
+ "pos_z": -58.4375,
+ "stations": "Toll Installation,Neumann Depot,Fossey Legacy,McArthur City,Chilton Port,Chu City,Thompson City,Lanier Depot"
+ },
+ {
+ "name": "Kurui Gubi",
+ "pos_x": -33.8125,
+ "pos_y": 39.34375,
+ "pos_z": -26.25,
+ "stations": "Campbell Prospect,Dedekind City,Petaja Dock,Lee Enterprise"
+ },
+ {
+ "name": "Kurui Xee",
+ "pos_x": -52.65625,
+ "pos_y": -46.375,
+ "pos_z": -133.78125,
+ "stations": "Cixin Dock,Huberath Laboratory,Cartmill Base"
+ },
+ {
+ "name": "Kuruidones",
+ "pos_x": 93.96875,
+ "pos_y": -111.6875,
+ "pos_z": -86.5,
+ "stations": "Rigaux Sanctuary,Parsons Landing,Safdie Enterprise"
+ },
+ {
+ "name": "Kurukana",
+ "pos_x": 40.96875,
+ "pos_y": -1.84375,
+ "pos_z": 77.90625,
+ "stations": "Coulomb Penitentiary,Hart Orbital,Czerny Landing,Bamford Holdings"
+ },
+ {
+ "name": "Kurukujiali",
+ "pos_x": 0.4375,
+ "pos_y": -59.4375,
+ "pos_z": 100.5625,
+ "stations": "Doi Works,Swigert Arena"
+ },
+ {
+ "name": "Kuruma",
+ "pos_x": -140.34375,
+ "pos_y": -55.59375,
+ "pos_z": -51.1875,
+ "stations": "Kneale Arena"
+ },
+ {
+ "name": "Kurumanit",
+ "pos_x": 146.40625,
+ "pos_y": 29.75,
+ "pos_z": -95.34375,
+ "stations": "Thompson Port,Levy Horizons"
+ },
+ {
+ "name": "Kurumbas",
+ "pos_x": 153.21875,
+ "pos_y": -31.59375,
+ "pos_z": -41.21875,
+ "stations": "Moffitt's Folly,de Balboa Horizons"
+ },
+ {
+ "name": "Kurumian",
+ "pos_x": 192.15625,
+ "pos_y": -180.9375,
+ "pos_z": -15.96875,
+ "stations": "Levi-Civita Hub,Horrocks Landing"
+ },
+ {
+ "name": "Kurundulli",
+ "pos_x": 58,
+ "pos_y": -77.40625,
+ "pos_z": 104.40625,
+ "stations": "Hay Beacon"
+ },
+ {
+ "name": "Kurung",
+ "pos_x": -137.3125,
+ "pos_y": 88.25,
+ "pos_z": -38.03125,
+ "stations": "Fawcett Dock"
+ },
+ {
+ "name": "Kurungagetu",
+ "pos_x": 10.6875,
+ "pos_y": -97.25,
+ "pos_z": 108.21875,
+ "stations": "Kurland Horizons,Helmholtz Station,Macarthur Colony"
+ },
+ {
+ "name": "Kurunggua",
+ "pos_x": 47.71875,
+ "pos_y": -123.1875,
+ "pos_z": 139.21875,
+ "stations": "Delsanti Enterprise,Wendelin Station,Koolhaas Prospect,Barbuy's Progress"
+ },
+ {
+ "name": "Kurunmatay",
+ "pos_x": 155.875,
+ "pos_y": -97.75,
+ "pos_z": -70.375,
+ "stations": "Brom Terminal,Jones Laboratory"
+ },
+ {
+ "name": "Kuruntabal",
+ "pos_x": 59.4375,
+ "pos_y": -99.5,
+ "pos_z": 60.8125,
+ "stations": "Brorsen Orbital,van Houten Survey,Niijima's Folly"
+ },
+ {
+ "name": "Kurunti",
+ "pos_x": -79.28125,
+ "pos_y": -44.125,
+ "pos_z": 63.4375,
+ "stations": "Al-Jazari Depot,Faris City,Illy Port"
+ },
+ {
+ "name": "Kurutas",
+ "pos_x": 57.84375,
+ "pos_y": 29.78125,
+ "pos_z": 99.96875,
+ "stations": "Lie Terminal,Tem Station,Hubble Settlement"
+ },
+ {
+ "name": "Kurutis",
+ "pos_x": -89.96875,
+ "pos_y": -44.09375,
+ "pos_z": -136.875,
+ "stations": "Sawyer Station"
+ },
+ {
+ "name": "Kurutsi",
+ "pos_x": 19.96875,
+ "pos_y": -63.78125,
+ "pos_z": -72.3125,
+ "stations": "Atiyah Horizons,Walter Hangar"
+ },
+ {
+ "name": "Kusang",
+ "pos_x": 110.625,
+ "pos_y": -63.5625,
+ "pos_z": 81.03125,
+ "stations": "Franklin Beacon,Oren Orbital,Bruce Observatory,Puiseux Vision,Meech Installation"
+ },
+ {
+ "name": "Kusat",
+ "pos_x": 144.71875,
+ "pos_y": 61.34375,
+ "pos_z": 46.0625,
+ "stations": "Ron Hubbard Hub,Julian Ring,Kronecker's Progress,Okorafor Installation"
+ },
+ {
+ "name": "Kusauts",
+ "pos_x": -18.3125,
+ "pos_y": -9.71875,
+ "pos_z": 121.84375,
+ "stations": "Tereshkova Camp,Thomas Stop"
+ },
+ {
+ "name": "Kusha",
+ "pos_x": 9.1875,
+ "pos_y": 63.28125,
+ "pos_z": 162.46875,
+ "stations": "Daimler Terminal,Elder Barracks,Sommerfeld Enterprise,Godel Vision"
+ },
+ {
+ "name": "Kushambo",
+ "pos_x": 104.6875,
+ "pos_y": -75.78125,
+ "pos_z": -61.3125,
+ "stations": "McKean Platform,Suntzeff Hangar,Meinel Base"
+ },
+ {
+ "name": "Kushen",
+ "pos_x": 26.46875,
+ "pos_y": -8.5,
+ "pos_z": 7.84375,
+ "stations": "Blalock Terminal,Malaspina Installation,Balmer Landing"
+ },
+ {
+ "name": "Kusubi Gong",
+ "pos_x": -102.375,
+ "pos_y": 36.59375,
+ "pos_z": 17.40625,
+ "stations": "Wittgenstein Refinery"
+ },
+ {
+ "name": "Kute",
+ "pos_x": 100.125,
+ "pos_y": -221.875,
+ "pos_z": 45.90625,
+ "stations": "Wafa Point"
+ },
+ {
+ "name": "Kutenis",
+ "pos_x": 116.46875,
+ "pos_y": 14.40625,
+ "pos_z": 13.59375,
+ "stations": "Legendre Terminal,Napier City,Karlsefni Park,Marques Hub,RenenBellot Vision,Bailey Orbital,Jones Orbital,Qian Relay,Vaez de Torres' Inheritance,Fremion Orbital"
+ },
+ {
+ "name": "Kutja",
+ "pos_x": -37.15625,
+ "pos_y": 77.71875,
+ "pos_z": 80.625,
+ "stations": "Moresby Installation,Weinbaum's Inheritance,Younghusband Penal colony"
+ },
+ {
+ "name": "Kutjalangai",
+ "pos_x": 48.4375,
+ "pos_y": -115.96875,
+ "pos_z": 58.59375,
+ "stations": "Chretien Oudemans City,Hawker Vision,Langley Terminal,Chawla Orbital,Zenbei Port,Hansteen Port"
+ },
+ {
+ "name": "Kutjalkini",
+ "pos_x": 98.5625,
+ "pos_y": -61.59375,
+ "pos_z": 101.125,
+ "stations": "Maire Landing,Furukawa Mines,Kawanishi Station"
+ },
+ {
+ "name": "Kutjang",
+ "pos_x": -171,
+ "pos_y": -49.75,
+ "pos_z": 16.125,
+ "stations": "Baraniecki Platform,Bunch Platform"
+ },
+ {
+ "name": "Kutjara",
+ "pos_x": 93.53125,
+ "pos_y": -57.84375,
+ "pos_z": -14.375,
+ "stations": "Suydam Depot"
+ },
+ {
+ "name": "Kutjarasvat",
+ "pos_x": -72.9375,
+ "pos_y": -60.4375,
+ "pos_z": -117.09375,
+ "stations": "Wittgenstein Landing,Musgrave Hub"
+ },
+ {
+ "name": "Kutjaricori",
+ "pos_x": 122.46875,
+ "pos_y": -0.84375,
+ "pos_z": -98.59375,
+ "stations": "Heinlein Orbital,Cernan Stop"
+ },
+ {
+ "name": "Kutjini",
+ "pos_x": -61,
+ "pos_y": 23.25,
+ "pos_z": -133.625,
+ "stations": "Macomb Arena"
+ },
+ {
+ "name": "Kutkha",
+ "pos_x": 60.59375,
+ "pos_y": -10.8125,
+ "pos_z": 17.15625,
+ "stations": "Macquorn Rankine Gateway,Ford Barracks,Ryazanski Station,Kregel Station,Chilton Orbital,Nagel Landing,Atwater Station,Kregel Relay,Hutton Dock,Harvey City"
+ },
+ {
+ "name": "Kutkhab",
+ "pos_x": -161.8125,
+ "pos_y": 23.875,
+ "pos_z": -13.03125,
+ "stations": "Khrenov Orbital"
+ },
+ {
+ "name": "Kutkinungs",
+ "pos_x": 146.90625,
+ "pos_y": -185.46875,
+ "pos_z": -32.90625,
+ "stations": "Resnik Survey,Mooz Survey,Lane Survey"
+ },
+ {
+ "name": "Kutnahin",
+ "pos_x": 88.90625,
+ "pos_y": -29.28125,
+ "pos_z": 100.09375,
+ "stations": "Lagadha Settlement"
+ },
+ {
+ "name": "Kutnal",
+ "pos_x": -87.4375,
+ "pos_y": -63.9375,
+ "pos_z": -89.78125,
+ "stations": "Oswald Point"
+ },
+ {
+ "name": "Kutnigi",
+ "pos_x": -16.5625,
+ "pos_y": -84.34375,
+ "pos_z": -59.53125,
+ "stations": "Redi Enterprise"
+ },
+ {
+ "name": "Kutnigin",
+ "pos_x": 110.46875,
+ "pos_y": -62.34375,
+ "pos_z": 98.625,
+ "stations": "Fairey Port,Shoujing Ring"
+ },
+ {
+ "name": "Kuukese",
+ "pos_x": -6.09375,
+ "pos_y": 5.65625,
+ "pos_z": 92.84375,
+ "stations": "Wingrove Port,Eisenstein Gateway,Jones Ring"
+ },
+ {
+ "name": "Kuuku",
+ "pos_x": 138.6875,
+ "pos_y": -83.375,
+ "pos_z": 60.5625,
+ "stations": "Rigaux Enterprise,Mainzer Gateway,Borrelly Enterprise"
+ },
+ {
+ "name": "Kuung Wa",
+ "pos_x": -15.59375,
+ "pos_y": -73.6875,
+ "pos_z": 121.3125,
+ "stations": "Darwin Dock"
+ },
+ {
+ "name": "Kuunggati",
+ "pos_x": -89.09375,
+ "pos_y": 33.34375,
+ "pos_z": -28.4375,
+ "stations": "Oramus Dock"
+ },
+ {
+ "name": "Kuungunitou",
+ "pos_x": -35.125,
+ "pos_y": -5.5625,
+ "pos_z": 140.46875,
+ "stations": "Furrer City"
+ },
+ {
+ "name": "KUV 410-4",
+ "pos_x": 9.96875,
+ "pos_y": 42.625,
+ "pos_z": -49.78125,
+ "stations": "Henderson Penal colony,Anderson Colony,Griffin Plant"
+ },
+ {
+ "name": "Kuwair",
+ "pos_x": 32.125,
+ "pos_y": 113.21875,
+ "pos_z": 91.78125,
+ "stations": "Thornycroft Terminal,Asire Horizons,Velho Installation,Zahn Horizons"
+ },
+ {
+ "name": "Kuwan Slavs",
+ "pos_x": -70.375,
+ "pos_y": -84.25,
+ "pos_z": -32.21875,
+ "stations": "Jemison Survey,Helms Platform"
+ },
+ {
+ "name": "Kuwema",
+ "pos_x": -4.09375,
+ "pos_y": -14.5,
+ "pos_z": -143.625,
+ "stations": "Schmitt Ring,Saberhagen Barracks,Al-Khalili Settlement,Napier Vision"
+ },
+ {
+ "name": "Kuwemaki",
+ "pos_x": 134.65625,
+ "pos_y": -226.90625,
+ "pos_z": -7.8125,
+ "stations": "The Jet's Hole,Dickinson Platform,Sitter Vision"
+ },
+ {
+ "name": "Kuwemarai",
+ "pos_x": 111.34375,
+ "pos_y": 64.09375,
+ "pos_z": -89.15625,
+ "stations": "Fourier Plant,Bombelli Settlement"
+ },
+ {
+ "name": "Kuwemargl",
+ "pos_x": 35.84375,
+ "pos_y": -43.625,
+ "pos_z": 77.15625,
+ "stations": "Baillaud Ring,Oosterhoff Vision,Pritchard Hub,Bok Survey,Zelazny Enterprise"
+ },
+ {
+ "name": "Kuwemasil",
+ "pos_x": 117.25,
+ "pos_y": 99.0625,
+ "pos_z": -40.5,
+ "stations": "Herzfeld City,Dutton Terminal,Krikalev Landing,Marques Relay"
+ },
+ {
+ "name": "Kuwembaa",
+ "pos_x": 159.5625,
+ "pos_y": 6.59375,
+ "pos_z": 4.28125,
+ "stations": "Gagnan Port,Runco Colony,Khrunov Hub,Henslow Silo,Francisco de Eliza Beacon"
+ },
+ {
+ "name": "Kuwembanda",
+ "pos_x": 89.1875,
+ "pos_y": -152.9375,
+ "pos_z": -61.5,
+ "stations": "Michalitsianos Estate,Goldberg Prospect,Hassanein's Progress"
+ },
+ {
+ "name": "Kuwembe",
+ "pos_x": 27.125,
+ "pos_y": 126.53125,
+ "pos_z": -107.25,
+ "stations": "Kingsbury Vision,Mackenzie Terminal,Morris Point"
+ },
+ {
+ "name": "Kuyu",
+ "pos_x": 89.3125,
+ "pos_y": -130.03125,
+ "pos_z": 9.75,
+ "stations": "Dugan Port"
+ },
+ {
+ "name": "Kvas",
+ "pos_x": 4.03125,
+ "pos_y": -18.3125,
+ "pos_z": 161.15625,
+ "stations": "Flynn Colony"
+ },
+ {
+ "name": "Kvash",
+ "pos_x": -94.40625,
+ "pos_y": 96.90625,
+ "pos_z": 82.21875,
+ "stations": "Wiley Station,Wilson Port,Bond Enterprise,Schlegel Gateway"
+ },
+ {
+ "name": "Kvashirua",
+ "pos_x": 44.3125,
+ "pos_y": -15.125,
+ "pos_z": -83.8125,
+ "stations": "Ray Refinery,Coelho Enterprise,Magnus Settlement"
+ },
+ {
+ "name": "Kvashodi",
+ "pos_x": 89.40625,
+ "pos_y": -30.5,
+ "pos_z": 137.34375,
+ "stations": "Felice Dock,Morris' Pride,Scheerbart Bastion"
+ },
+ {
+ "name": "Kwakimo",
+ "pos_x": 62.0625,
+ "pos_y": -58,
+ "pos_z": -79.5625,
+ "stations": "Khan Enterprise,Silves Base,Sinclair Enterprise"
+ },
+ {
+ "name": "Kwakinne",
+ "pos_x": -179.34375,
+ "pos_y": 21.75,
+ "pos_z": -11.9375,
+ "stations": "Mourelle Terminal,Popper Platform,Wrangel Horizons"
+ },
+ {
+ "name": "Kwakiutl",
+ "pos_x": 117.75,
+ "pos_y": 100.75,
+ "pos_z": 124.34375,
+ "stations": "Lavrador Point,Alten Mines,Crook Mines"
+ },
+ {
+ "name": "Kwakw",
+ "pos_x": 139.75,
+ "pos_y": -88.65625,
+ "pos_z": 71.375,
+ "stations": "Penrose Reach"
+ },
+ {
+ "name": "Kwakwakwal",
+ "pos_x": 19.9375,
+ "pos_y": 23.71875,
+ "pos_z": 135.25,
+ "stations": "Thagard Station,Ivanchenkov City,Schirra Hub"
+ },
+ {
+ "name": "Kwamindjara",
+ "pos_x": 117.34375,
+ "pos_y": -198.625,
+ "pos_z": 15.40625,
+ "stations": "Paczynski Station,Flaugergues Platform,Danforth Prospect,Lane Depot"
+ },
+ {
+ "name": "Kwangatjali",
+ "pos_x": 29.625,
+ "pos_y": 6.46875,
+ "pos_z": 110.75,
+ "stations": "Erikson Station,Lynn Refinery,Vaez de Torres' Progress"
+ },
+ {
+ "name": "Kwangwutii",
+ "pos_x": -8.90625,
+ "pos_y": -2.375,
+ "pos_z": -122.28125,
+ "stations": "Boulton City,Overmyer Ring,Whitney Hub"
+ },
+ {
+ "name": "Kwanteru",
+ "pos_x": 123.375,
+ "pos_y": 50.53125,
+ "pos_z": -0.28125,
+ "stations": "Tereshkova Landing,Popovich Settlement,Locke Barracks"
+ },
+ {
+ "name": "Kwarandji",
+ "pos_x": 16.03125,
+ "pos_y": -212.03125,
+ "pos_z": 12.5625,
+ "stations": "Edwards Point,Maury Keep"
+ },
+ {
+ "name": "Kwaraseti",
+ "pos_x": -22.9375,
+ "pos_y": -3.5,
+ "pos_z": -91.15625,
+ "stations": "Moore Holdings,Pierce Hub"
+ },
+ {
+ "name": "Kwarata",
+ "pos_x": 174,
+ "pos_y": -131.34375,
+ "pos_z": 75.28125,
+ "stations": "Hughes Enterprise,Nahavandi Enterprise,Grigorovich Vision,Hausdorff Base"
+ },
+ {
+ "name": "Kwaritreni",
+ "pos_x": -21.65625,
+ "pos_y": 27.21875,
+ "pos_z": -142.34375,
+ "stations": "Banks Hub,Danvers Settlement,Thagard Depot,Balmer Platform"
+ },
+ {
+ "name": "Kwatee",
+ "pos_x": -41,
+ "pos_y": -51.40625,
+ "pos_z": 167.53125,
+ "stations": "Herreshoff Gateway,Smith Dock,Cartier Dock,Barcelo Works"
+ },
+ {
+ "name": "Kwati",
+ "pos_x": 88.3125,
+ "pos_y": -182.59375,
+ "pos_z": 104.90625,
+ "stations": "Valz Outpost,Gustav Sporer Port,Benford's Inheritance"
+ },
+ {
+ "name": "Kwatiles",
+ "pos_x": -44.3125,
+ "pos_y": 28.59375,
+ "pos_z": 63.21875,
+ "stations": "Elion Works"
+ },
+ {
+ "name": "Kwatis",
+ "pos_x": 60.65625,
+ "pos_y": -166.84375,
+ "pos_z": 150.09375,
+ "stations": "Hale Base,Furuta Mines"
+ },
+ {
+ "name": "Kwatkwala",
+ "pos_x": -140.84375,
+ "pos_y": -136.0625,
+ "pos_z": -17.90625,
+ "stations": "Ayerdhal Dock"
+ },
+ {
+ "name": "Kwatsu",
+ "pos_x": 142.40625,
+ "pos_y": -8.65625,
+ "pos_z": -3.8125,
+ "stations": "Pascal Landing,Benford Hub,Fossum Ring"
+ },
+ {
+ "name": "Kwattrages",
+ "pos_x": 81.875,
+ "pos_y": -2.5625,
+ "pos_z": -3.21875,
+ "stations": "Roentgen Port,Ampere Enterprise,Mach Station"
+ },
+ {
+ "name": "Kwatyri",
+ "pos_x": -119.875,
+ "pos_y": 89.0625,
+ "pos_z": 37.46875,
+ "stations": "Steakley Landing"
+ },
+ {
+ "name": "Kwatyriel",
+ "pos_x": -139.9375,
+ "pos_y": 90.0625,
+ "pos_z": -31.75,
+ "stations": "Lasswitz Installation,Sellings Legacy,Shipton's Exile,Friend Relay"
+ },
+ {
+ "name": "Kwazahui",
+ "pos_x": 75.875,
+ "pos_y": 5.8125,
+ "pos_z": 20.90625,
+ "stations": "Watts Terminal,Baudry Gateway,Kagan Barracks"
+ },
+ {
+ "name": "Kwekwaki",
+ "pos_x": 90.34375,
+ "pos_y": 20.34375,
+ "pos_z": -39.78125,
+ "stations": "Somerset City,Anderson Terminal,Al-Battani City,Killough's Folly"
+ },
+ {
+ "name": "Kwekwatta",
+ "pos_x": 19.875,
+ "pos_y": 157.59375,
+ "pos_z": -4.9375,
+ "stations": "Tombaugh Colony"
+ },
+ {
+ "name": "Kwekwaxawe",
+ "pos_x": 100.5,
+ "pos_y": 63.84375,
+ "pos_z": -0.28125,
+ "stations": "Chorel Hub,Laplace Hangar,Ali Port,Marusek Terminal"
+ },
+ {
+ "name": "Kwele",
+ "pos_x": -159.875,
+ "pos_y": -10.625,
+ "pos_z": 73.71875,
+ "stations": "Wallin Vision,Gibbs Vision,Britnev Vision,Laird Legacy,Lobachevsky Settlement"
+ },
+ {
+ "name": "Kwelegera",
+ "pos_x": 158.34375,
+ "pos_y": -163.1875,
+ "pos_z": 71.75,
+ "stations": "Axon Terminal,Qushji Platform,Faye Port,Russell Beacon"
+ },
+ {
+ "name": "Kwelegus",
+ "pos_x": 75.15625,
+ "pos_y": -161.46875,
+ "pos_z": 65.71875,
+ "stations": "Zenbei Depot,Roche Installation"
+ },
+ {
+ "name": "Kwelenii",
+ "pos_x": 23.09375,
+ "pos_y": -192.09375,
+ "pos_z": 76.4375,
+ "stations": "Dilworth Terminal,Shklovsky Hub,Johnson Hub,Whitcomb Market,Harrington Dock,Banks Settlement,Sato Hub,Dedekind Horizons,Heinemann Orbital"
+ },
+ {
+ "name": "Kwelepita",
+ "pos_x": 150.59375,
+ "pos_y": 23.09375,
+ "pos_z": -5.9375,
+ "stations": "Krylov Outpost,Anthony Hub"
+ },
+ {
+ "name": "Kweleutahe",
+ "pos_x": 18.09375,
+ "pos_y": -5.28125,
+ "pos_z": 89.46875,
+ "stations": "Merchiston Dock,Samuda Depot,Bernard Relay"
+ },
+ {
+ "name": "Kwer",
+ "pos_x": -79.4375,
+ "pos_y": 15.25,
+ "pos_z": -154.875,
+ "stations": "Kononenko Port,Coleman Depot,Gerlache Base"
+ },
+ {
+ "name": "Kweretet",
+ "pos_x": 36,
+ "pos_y": 41.5,
+ "pos_z": -84.5625,
+ "stations": "Bennett Settlement"
+ },
+ {
+ "name": "Kweretiansa",
+ "pos_x": 20.53125,
+ "pos_y": -3.5625,
+ "pos_z": -175,
+ "stations": "MacVicar Camp,Busch Refinery"
+ },
+ {
+ "name": "Kwiambe",
+ "pos_x": 98.25,
+ "pos_y": -249.21875,
+ "pos_z": 19.53125,
+ "stations": "Clifford Base,Guth Vision,Foster Port"
+ },
+ {
+ "name": "Kwiambilara",
+ "pos_x": 148.0625,
+ "pos_y": -119.625,
+ "pos_z": 50.15625,
+ "stations": "Lambert Terminal,Andree Terminal,Taylor Terminal,Donati Dock,Sheremetevsky Dock"
+ },
+ {
+ "name": "Kwiambojawa",
+ "pos_x": -118.4375,
+ "pos_y": -35.3125,
+ "pos_z": -36.78125,
+ "stations": "Ostrander's Claim,Selberg Prospect"
+ },
+ {
+ "name": "Kwiamo",
+ "pos_x": 24.90625,
+ "pos_y": 22.71875,
+ "pos_z": -122.96875,
+ "stations": "Abernathy Plant"
+ },
+ {
+ "name": "Kwiamong",
+ "pos_x": -41.5,
+ "pos_y": 9.5,
+ "pos_z": -135.4375,
+ "stations": "Mallett Gateway,Ron Hubbard Station,Leckie Colony,Santarem Keep"
+ },
+ {
+ "name": "Kwiamoni",
+ "pos_x": -40,
+ "pos_y": -116.9375,
+ "pos_z": -122.78125,
+ "stations": "Amnuel Dock,Jiushao Dock"
+ },
+ {
+ "name": "Kwikumat",
+ "pos_x": 199.21875,
+ "pos_y": -108.40625,
+ "pos_z": -0.8125,
+ "stations": "Mukai Relay"
+ },
+ {
+ "name": "Kwin",
+ "pos_x": 114.5,
+ "pos_y": -63.9375,
+ "pos_z": -83.59375,
+ "stations": "Bolger Settlement,Bridger Settlement"
+ },
+ {
+ "name": "Ky Lin",
+ "pos_x": -140.125,
+ "pos_y": 60.90625,
+ "pos_z": 88.21875,
+ "stations": "Flynn Landing,Pinto Prospect"
+ },
+ {
+ "name": "Kyamatae",
+ "pos_x": 103.125,
+ "pos_y": 89.59375,
+ "pos_z": 40.75,
+ "stations": "Lysenko Enterprise,Coleman Terminal,Smith Vision,Morgan's Progress,Tolstoi's Inheritance"
+ },
+ {
+ "name": "KZ Andromedae",
+ "pos_x": -72.65625,
+ "pos_y": -15.3125,
+ "pos_z": -21.34375,
+ "stations": "Snodgrass Survey,Linaweaver Terminal,Wyeth Camp,Holberg Vision"
+ },
+ {
+ "name": "L 119-33",
+ "pos_x": 25.1875,
+ "pos_y": -43.375,
+ "pos_z": 34.34375,
+ "stations": "Oersted Dock,den Berg Refinery"
+ },
+ {
+ "name": "L 12-19",
+ "pos_x": 113.78125,
+ "pos_y": -85.96875,
+ "pos_z": 56.53125,
+ "stations": "Bolton Mines"
+ },
+ {
+ "name": "L 12-40",
+ "pos_x": 88.125,
+ "pos_y": -65.6875,
+ "pos_z": 47.375,
+ "stations": "Abbot Enterprise,August von Steinheil Platform,Elwood Point"
+ },
+ {
+ "name": "L 170-63",
+ "pos_x": 83.625,
+ "pos_y": -172.5,
+ "pos_z": 61.125,
+ "stations": "Gaughan Terminal,Rechtin Ring,Engle City,Helffrich Observatory,Akers' Folly,Myasishchev Point,Sato Stop"
+ },
+ {
+ "name": "L 190-21",
+ "pos_x": 35.375,
+ "pos_y": 0.1875,
+ "pos_z": 7.75,
+ "stations": "Gurragchaa Orbital,Arkwright Vision,Bondar Terminal,Lockhart Holdings,Wescott Port,Dirichlet Point,Bamford City,Aleksandrov Port"
+ },
+ {
+ "name": "L 206-187",
+ "pos_x": 17.375,
+ "pos_y": -16.25,
+ "pos_z": 40.65625,
+ "stations": "Tarelkin Station,Bhabha Orbital,McCaffrey Escape"
+ },
+ {
+ "name": "L 214-72",
+ "pos_x": 37.53125,
+ "pos_y": -95.15625,
+ "pos_z": 61.71875,
+ "stations": "Nomura Landing"
+ },
+ {
+ "name": "L 258-146",
+ "pos_x": 33.84375,
+ "pos_y": 6.25,
+ "pos_z": 29.21875,
+ "stations": "Tito Camp,Mendeleev Hangar,Jones' Progress"
+ },
+ {
+ "name": "L 26-27",
+ "pos_x": 19.40625,
+ "pos_y": -21.53125,
+ "pos_z": 14.90625,
+ "stations": "McCoy City,Aldrin Colony,Chretien Colony,Citi Relay"
+ },
+ {
+ "name": "L 286-71",
+ "pos_x": 32.625,
+ "pos_y": -113.53125,
+ "pos_z": 65.125,
+ "stations": "Frost Refinery,Lynden-Bell Installation"
+ },
+ {
+ "name": "L 639-45",
+ "pos_x": -11.34375,
+ "pos_y": -26.25,
+ "pos_z": 39.0625,
+ "stations": "Walker Orbital,Malzberg Hub"
+ },
+ {
+ "name": "L 93-11",
+ "pos_x": 105.625,
+ "pos_y": -76.59375,
+ "pos_z": 11.4375,
+ "stations": "Merrill Depot,Malvern Mine,Oikawa Platform"
+ },
+ {
+ "name": "La Rochelle",
+ "pos_x": 2.03125,
+ "pos_y": -11.8125,
+ "pos_z": 10.8125,
+ "stations": "Shaver Dock,Wait Colburn Vision"
+ },
+ {
+ "name": "La Te Ti",
+ "pos_x": -105.03125,
+ "pos_y": -34.4375,
+ "pos_z": -71.46875,
+ "stations": "Glenn City,Kroehl Survey,Mohun Beacon"
+ },
+ {
+ "name": "La Tenha",
+ "pos_x": -4.15625,
+ "pos_y": 91,
+ "pos_z": 49.59375,
+ "stations": "Rozhdestvensky Horizons,Williams Dock,Kraepelin Dock,Vlamingh Settlement,Clair Prospect,Fibonacci's Progress"
+ },
+ {
+ "name": "La Thixo",
+ "pos_x": 187.21875,
+ "pos_y": -163.25,
+ "pos_z": -6.84375,
+ "stations": "Becvar Dock,Farmer Hub"
+ },
+ {
+ "name": "Labe",
+ "pos_x": -17.34375,
+ "pos_y": 12.46875,
+ "pos_z": -68.59375,
+ "stations": "Hermite Terminal,Drew Settlement,den Berg Mines"
+ },
+ {
+ "name": "Labed",
+ "pos_x": -86.875,
+ "pos_y": 32.3125,
+ "pos_z": -59.90625,
+ "stations": "Carson Survey,Midgley Colony"
+ },
+ {
+ "name": "Labera",
+ "pos_x": 121.09375,
+ "pos_y": -197.65625,
+ "pos_z": -2.4375,
+ "stations": "Paul Mines"
+ },
+ {
+ "name": "Lacab Kui",
+ "pos_x": 46.1875,
+ "pos_y": -87.0625,
+ "pos_z": 40.3125,
+ "stations": "Kandrup Colony,Shunkai Mines,Trumpler Installation,Suri Terminal"
+ },
+ {
+ "name": "Lacabil",
+ "pos_x": 149.9375,
+ "pos_y": -160.5625,
+ "pos_z": 99.84375,
+ "stations": "Kerimov Dock"
+ },
+ {
+ "name": "Lacaille 8760",
+ "pos_x": -0.6875,
+ "pos_y": -9.09375,
+ "pos_z": 9.09375,
+ "stations": "Reilly Beacon,Serebrov City,Chiao Landing"
+ },
+ {
+ "name": "Lacaille 9352",
+ "pos_x": -0.40625,
+ "pos_y": -9.8125,
+ "pos_z": 4.21875,
+ "stations": "Gupta City,Kirtley Platform,Rasch Hub"
+ },
+ {
+ "name": "Lacani",
+ "pos_x": 103.65625,
+ "pos_y": -44.53125,
+ "pos_z": 100.28125,
+ "stations": "Bolkow Orbital,Williams Prospect,Karlsefni Terminal,Finlay-Freundlich City,Regiomontanus Hub"
+ },
+ {
+ "name": "Lacans",
+ "pos_x": 134.8125,
+ "pos_y": -138.5,
+ "pos_z": -25.125,
+ "stations": "Wilson Orbital,al-Din al-Urdi Dock,Jordan Survey"
+ },
+ {
+ "name": "Lachangu",
+ "pos_x": 57.875,
+ "pos_y": -69.125,
+ "pos_z": 31.90625,
+ "stations": "Adelman Dock,Ruppelt Station"
+ },
+ {
+ "name": "Lachazi",
+ "pos_x": -115.3125,
+ "pos_y": 28.6875,
+ "pos_z": -50.375,
+ "stations": "Linge Hub,Hamilton Dock,Cook Outpost"
+ },
+ {
+ "name": "Lachelka",
+ "pos_x": 69.6875,
+ "pos_y": 40.75,
+ "pos_z": 117.53125,
+ "stations": "Ferguson Survey,Usachov Point"
+ },
+ {
+ "name": "Lactona",
+ "pos_x": -48.28125,
+ "pos_y": -113.34375,
+ "pos_z": -6.59375,
+ "stations": "Nowak Beacon,Lee's Progress,Olivas Landing"
+ },
+ {
+ "name": "Lactonacaya",
+ "pos_x": 41.53125,
+ "pos_y": -105.65625,
+ "pos_z": -48.8125,
+ "stations": "Lavochkin Terminal,Marsden Enterprise,Shute Mines,Rosse Hub"
+ },
+ {
+ "name": "Lactonaz",
+ "pos_x": -73.15625,
+ "pos_y": -121.53125,
+ "pos_z": 27.96875,
+ "stations": "vo Dock,Birkhoff Hub,Szentmartony Prospect"
+ },
+ {
+ "name": "Lactora",
+ "pos_x": -91.03125,
+ "pos_y": -133.90625,
+ "pos_z": 74.8125,
+ "stations": "Kafka Settlement,Kirtley Station,Cummings Penal colony"
+ },
+ {
+ "name": "Lactorma",
+ "pos_x": 99.40625,
+ "pos_y": 40.65625,
+ "pos_z": -69.25,
+ "stations": "Cleve Holdings,LeConte Port,Zebrowski Landing"
+ },
+ {
+ "name": "Ladabalbasa",
+ "pos_x": 59.71875,
+ "pos_y": -11.34375,
+ "pos_z": -7.3125,
+ "stations": "Malyshev Oasis,Al Saud Gateway"
+ },
+ {
+ "name": "Ladagao",
+ "pos_x": 128,
+ "pos_y": -248.59375,
+ "pos_z": 90.4375,
+ "stations": "Herbig Reformatory,Wolszczan Plant"
+ },
+ {
+ "name": "Ladakhi",
+ "pos_x": 115.8125,
+ "pos_y": -237.78125,
+ "pos_z": -17.34375,
+ "stations": "Al-Khujandi Landing,Brouwer Outpost,Whitcomb Point"
+ },
+ {
+ "name": "Ladon",
+ "pos_x": -17.46875,
+ "pos_y": -59.84375,
+ "pos_z": -52.59375,
+ "stations": "Weitz Refinery,Barreiros Base"
+ },
+ {
+ "name": "Laedla",
+ "pos_x": 78.46875,
+ "pos_y": -91.3125,
+ "pos_z": 29.375,
+ "stations": "Kummer Acropolis,Kludze Colony,Gusmao Prospect,Fan Station,Barnes Town,Staus Palace"
+ },
+ {
+ "name": "Lafqueela",
+ "pos_x": 77.84375,
+ "pos_y": -33.5,
+ "pos_z": -13.25,
+ "stations": "Grant's Progress,Stott Enterprise"
+ },
+ {
+ "name": "Lafquene",
+ "pos_x": -84.375,
+ "pos_y": -141.03125,
+ "pos_z": -11.78125,
+ "stations": "Reed Landing,Kazantsev Platform"
+ },
+ {
+ "name": "Laga",
+ "pos_x": -31.78125,
+ "pos_y": 94.78125,
+ "pos_z": -116.875,
+ "stations": "Reynolds City,Ledyard Ring,Vancouver Hub,Snodgrass Arsenal"
+ },
+ {
+ "name": "Lagalas",
+ "pos_x": -104.78125,
+ "pos_y": -60.71875,
+ "pos_z": 98.15625,
+ "stations": "Feustel Point,Weierstrass Settlement"
+ },
+ {
+ "name": "Lagar",
+ "pos_x": 75.71875,
+ "pos_y": -195.5,
+ "pos_z": 123.96875,
+ "stations": "Ban Vision,Charlois Dock,McCool Landing,Rond d'Alembert Works,Lukyanenko Prospect"
+ },
+ {
+ "name": "Lagay",
+ "pos_x": 95.59375,
+ "pos_y": 81.3125,
+ "pos_z": 75.625,
+ "stations": "Noli Prospect,Pavlou Outpost"
+ },
+ {
+ "name": "Lagoon Sector FW-W d1-122",
+ "pos_x": -467.75,
+ "pos_y": -93.1875,
+ "pos_z": 4485.625,
+ "stations": "Attenborough's Watch"
+ },
+ {
+ "name": "Lagoon Sector NI-S b4-10",
+ "pos_x": -469.1875,
+ "pos_y": -84.84375,
+ "pos_z": 4456.125,
+ "stations": "Amundsen Terminal"
+ },
+ {
+ "name": "Lagunnosso",
+ "pos_x": -36.25,
+ "pos_y": 90.6875,
+ "pos_z": -18.8125,
+ "stations": "Bulgarin Terminal,Wilkes Survey,Besonders Vision,Pond Survey"
+ },
+ {
+ "name": "Laguts",
+ "pos_x": 87,
+ "pos_y": 110,
+ "pos_z": -96.09375,
+ "stations": "Casper Hub,Mach Vision,Durrance Hub,Noakes Dock,Oltion Prospect,Hambly Penal colony"
+ },
+ {
+ "name": "Laguvii",
+ "pos_x": 39.59375,
+ "pos_y": -28.90625,
+ "pos_z": 156.6875,
+ "stations": "Barbosa Dock,Norman Base"
+ },
+ {
+ "name": "Lahara",
+ "pos_x": 68.40625,
+ "pos_y": -142.90625,
+ "pos_z": -58.90625,
+ "stations": "Urata Hub,Watson Relay,Drake Barracks,Dugan Terminal"
+ },
+ {
+ "name": "Lahasim",
+ "pos_x": -35.21875,
+ "pos_y": 141.0625,
+ "pos_z": 33.5,
+ "stations": "Beaufoy Reach"
+ },
+ {
+ "name": "Lahau",
+ "pos_x": -15.375,
+ "pos_y": -115.1875,
+ "pos_z": 128.96875,
+ "stations": "Abe Orbital"
+ },
+ {
+ "name": "Lahaua",
+ "pos_x": 82.78125,
+ "pos_y": -181.65625,
+ "pos_z": 118.59375,
+ "stations": "Aaronson Orbital,Boscovich Station,Sorayama Platform,Brendan Refinery,Lefschetz Plant"
+ },
+ {
+ "name": "Lahaunai",
+ "pos_x": 97.84375,
+ "pos_y": 1,
+ "pos_z": 120.03125,
+ "stations": "Kingsmill's Claim"
+ },
+ {
+ "name": "Lahu",
+ "pos_x": 147.3125,
+ "pos_y": 57.3125,
+ "pos_z": 71.75,
+ "stations": "Klein's Exile,Stuart Vision"
+ },
+ {
+ "name": "Lahua",
+ "pos_x": -79.4375,
+ "pos_y": 39.46875,
+ "pos_z": -80.125,
+ "stations": "Sanger Horizons,Lorenz Works,Russell Plant"
+ },
+ {
+ "name": "Lahun",
+ "pos_x": 114.84375,
+ "pos_y": -154.125,
+ "pos_z": -25.4375,
+ "stations": "Barbuy Enterprise,Goodman Relay,Schaumasse Camp"
+ },
+ {
+ "name": "Lai Shangu",
+ "pos_x": 43.96875,
+ "pos_y": -44.5625,
+ "pos_z": 154.84375,
+ "stations": "Martiniere's Pride,Ikeya Reformatory"
+ },
+ {
+ "name": "Lai Shas",
+ "pos_x": -71.71875,
+ "pos_y": -40.09375,
+ "pos_z": 166.8125,
+ "stations": "Morris Outpost,Vlamingh Arena"
+ },
+ {
+ "name": "Lai Shui",
+ "pos_x": 141.34375,
+ "pos_y": -100.3125,
+ "pos_z": 80.1875,
+ "stations": "Wenzel Port"
+ },
+ {
+ "name": "Laiawa",
+ "pos_x": 43.5,
+ "pos_y": 34.625,
+ "pos_z": 59.78125,
+ "stations": "Utley Base,Viktorenko Dock,Camarda Dock,Morgan Prospect,Holden Enterprise"
+ },
+ {
+ "name": "Laiawawal",
+ "pos_x": -60.59375,
+ "pos_y": 65.90625,
+ "pos_z": -27.78125,
+ "stations": "Roberts Port,Fernao do Po Camp,Crowley Keep"
+ },
+ {
+ "name": "Laifan",
+ "pos_x": -96.1875,
+ "pos_y": -106.875,
+ "pos_z": 66.84375,
+ "stations": "Onizuka Ring,Chilton Dock,Kidman Prospect,Busch Survey,Abbott Installation"
+ },
+ {
+ "name": "Laifang",
+ "pos_x": -97.125,
+ "pos_y": 70.1875,
+ "pos_z": 84.03125,
+ "stations": "Clerk Camp"
+ },
+ {
+ "name": "Laifangyi",
+ "pos_x": 53.65625,
+ "pos_y": -16.75,
+ "pos_z": 0.6875,
+ "stations": "Anders Hub,Johnson Hub,Zalyotin Hub,Lovelace City,Anning Enterprise"
+ },
+ {
+ "name": "Laiman",
+ "pos_x": -95.34375,
+ "pos_y": 116.5625,
+ "pos_z": 125.15625,
+ "stations": "Kirk's Claim"
+ },
+ {
+ "name": "Lak",
+ "pos_x": 21.15625,
+ "pos_y": -73.375,
+ "pos_z": 71.03125,
+ "stations": "Sabine Landing,Celsius Dock,Korolev Prospect,Carroll Vision"
+ },
+ {
+ "name": "Laka",
+ "pos_x": 110.40625,
+ "pos_y": -234.53125,
+ "pos_z": 115.09375,
+ "stations": "The Shoulder Of Orion,AdelaidePoonToon,Fadlan Point,Hardy Vista,Bellamy Gateway"
+ },
+ {
+ "name": "Lakhant",
+ "pos_x": 64.71875,
+ "pos_y": 6.875,
+ "pos_z": 122.3125,
+ "stations": "Akiyama Mines,Chretien Arena"
+ },
+ {
+ "name": "Lakheo",
+ "pos_x": 15.71875,
+ "pos_y": 3.03125,
+ "pos_z": -116.96875,
+ "stations": "Leeuwenhoek Port,Pierres Barracks"
+ },
+ {
+ "name": "Lakho",
+ "pos_x": -18.875,
+ "pos_y": 65.625,
+ "pos_z": -118.125,
+ "stations": "Ledyard Gateway,Stjepan Seljan Enterprise,Phillips Base,Littlewood Base"
+ },
+ {
+ "name": "Laklang",
+ "pos_x": 17.625,
+ "pos_y": -47.375,
+ "pos_z": 79.5,
+ "stations": "Great Point,Ahmed Installation"
+ },
+ {
+ "name": "Laklano",
+ "pos_x": -126.71875,
+ "pos_y": 126.59375,
+ "pos_z": -42.5625,
+ "stations": "Morris Oasis,Sylvester Oasis"
+ },
+ {
+ "name": "Lakluit",
+ "pos_x": -101.6875,
+ "pos_y": 25.125,
+ "pos_z": -14.0625,
+ "stations": "Garriott Hub,Zalyotin Park,Wittgenstein Ring,Payne Barracks,Steinmuller Base"
+ },
+ {
+ "name": "Lakluita",
+ "pos_x": 75,
+ "pos_y": -99.34375,
+ "pos_z": 78.125,
+ "stations": "Hill Tinsley Dock,Filippenko Terminal,Fabian Point,Kagawa Escape,Nelson Point,Cannon Lab,Ohain Installation,Drake's Inheritance,Jackson Prospect"
+ },
+ {
+ "name": "Lakota",
+ "pos_x": -8.15625,
+ "pos_y": 49.0625,
+ "pos_z": 26.21875,
+ "stations": "Shawn Zaman,Winnard's Hole,Vela Gateway,Maler Park,Volterra Lab"
+ },
+ {
+ "name": "Laksak",
+ "pos_x": -21.53125,
+ "pos_y": -6.3125,
+ "pos_z": 116.03125,
+ "stations": "Trader's Rest,Stjepan Seljan Hub,Littlewood Gateway,Kennan Installation,West City,Bugrov Dock,Pinto City,Rotsler Station,Reisman Vision,Hughes Settlement,Hedin Hub,Sekelj Port,Laumer City,Malcolm Enterprise,Scalzi Point"
+ },
+ {
+ "name": "Laksar",
+ "pos_x": 49.71875,
+ "pos_y": -93.125,
+ "pos_z": -103.78125,
+ "stations": "Mandel Port,Molyneux Hub,Ayers Ring,Barnaby Terminal"
+ },
+ {
+ "name": "Laksara",
+ "pos_x": -78.25,
+ "pos_y": 99.25,
+ "pos_z": -73.34375,
+ "stations": "Joliot-Curie Platform,Matteucci Port"
+ },
+ {
+ "name": "Laksha",
+ "pos_x": 169.8125,
+ "pos_y": -71.71875,
+ "pos_z": 50.96875,
+ "stations": "Safdie Platform,Aguirre Installation,Luther Survey,Chawla Landing"
+ },
+ {
+ "name": "Lakshalita",
+ "pos_x": -129.75,
+ "pos_y": -65.375,
+ "pos_z": -79.46875,
+ "stations": "Adams Survey"
+ },
+ {
+ "name": "Lakshma",
+ "pos_x": -152.1875,
+ "pos_y": -10.28125,
+ "pos_z": 4.3125,
+ "stations": "Chiang Terminal,Spielberg Orbital,Champlain Installation,Rey Port"
+ },
+ {
+ "name": "Lakshu",
+ "pos_x": -163.0625,
+ "pos_y": 2.4375,
+ "pos_z": 89.34375,
+ "stations": "Taylor Dock,Clifton Holdings,Plante City,Velho Hub"
+ },
+ {
+ "name": "Laksmi",
+ "pos_x": 43.84375,
+ "pos_y": -218.375,
+ "pos_z": -41.34375,
+ "stations": "Hume Survey,Albitzky Outpost"
+ },
+ {
+ "name": "Laksmii",
+ "pos_x": -35.84375,
+ "pos_y": -121.125,
+ "pos_z": -63.46875,
+ "stations": "Koishikawa's Claim"
+ },
+ {
+ "name": "Lakurbal",
+ "pos_x": -102.0625,
+ "pos_y": 25.6875,
+ "pos_z": 98.6875,
+ "stations": "Schrodinger City,Kraepelin City,Shelley Landing,Molina Hub"
+ },
+ {
+ "name": "Lakuxi",
+ "pos_x": 103.28125,
+ "pos_y": 57.4375,
+ "pos_z": -112.15625,
+ "stations": "Temple Arena"
+ },
+ {
+ "name": "Lalande 10797",
+ "pos_x": -8.375,
+ "pos_y": 6.1875,
+ "pos_z": -70.53125,
+ "stations": "Hippalus Gateway,Williams Hub,Anthony Legacy,Neff Hub,Jemison Enterprise,Steakley Terminal,Plexico Gateway,Ross Station,Hendel Gateway,Sanger Base,Napier Laboratory,Gloss Hub,Gibson Ring,Larson Installation,Lindbohm Hub"
+ },
+ {
+ "name": "Lalande 11206",
+ "pos_x": -64.5,
+ "pos_y": 45.625,
+ "pos_z": -97.96875,
+ "stations": "Kovalyonok Gateway,Pawelczyk Station,Cavendish Hub,Cadamosto Hub"
+ },
+ {
+ "name": "Lalande 13198",
+ "pos_x": 53.6875,
+ "pos_y": 0.25,
+ "pos_z": -80.5625,
+ "stations": "Bramah City,Bresnik Orbital,Stevin Depot,Malerba Enterprise,Furukawa Works"
+ },
+ {
+ "name": "Lalande 13942",
+ "pos_x": -8.21875,
+ "pos_y": 22.46875,
+ "pos_z": -49.625,
+ "stations": "Diesel Enterprise,Fourneyron Installation"
+ },
+ {
+ "name": "Lalande 14146",
+ "pos_x": 77.875,
+ "pos_y": -0.3125,
+ "pos_z": -71,
+ "stations": "Haisheng City,Babbage Terminal"
+ },
+ {
+ "name": "Lalande 15394",
+ "pos_x": 23.6875,
+ "pos_y": 26.25,
+ "pos_z": -57.46875,
+ "stations": "Salam Station,Pailes Station,Ali Barracks,Lister Mines,Malcolm Lab"
+ },
+ {
+ "name": "Lalande 15547",
+ "pos_x": 25.46875,
+ "pos_y": 32.125,
+ "pos_z": -65.625,
+ "stations": "McDevitt Mines"
+ },
+ {
+ "name": "Lalande 16904",
+ "pos_x": -31.46875,
+ "pos_y": 80,
+ "pos_z": -97.8125,
+ "stations": "Gagnan Hub,McArthur Station,Galilei Terminal,Lopez de Legazpi Point"
+ },
+ {
+ "name": "Lalande 17161",
+ "pos_x": 0.03125,
+ "pos_y": 54.46875,
+ "pos_z": -67.53125,
+ "stations": "Ford Plant"
+ },
+ {
+ "name": "Lalande 1799",
+ "pos_x": -28.8125,
+ "pos_y": -58.09375,
+ "pos_z": -23.5,
+ "stations": "Teller Ring,Watson Terminal,Cramer Installation"
+ },
+ {
+ "name": "Lalande 18115",
+ "pos_x": -3.84375,
+ "pos_y": 14,
+ "pos_z": -14.40625,
+ "stations": "Preuss Orbital,Waldeck Terminal,Lawhead Gateway,Robinson Hub,McMullen Hub,Steiner Installation,Ziemkiewicz Hub,Karlsefni Gateway,Garay Base,Guin Dock,Gunn Station,Greenleaf Base"
+ },
+ {
+ "name": "Lalande 21927",
+ "pos_x": -63.96875,
+ "pos_y": 109.46875,
+ "pos_z": -64.34375,
+ "stations": "Hilbert Base,Janes Bastion,Henderson Vision"
+ },
+ {
+ "name": "Lalande 22701",
+ "pos_x": 52.84375,
+ "pos_y": 90.46875,
+ "pos_z": 10.46875,
+ "stations": "Laval Port,Khrenov Installation,Hutton Ring,Deere Dock,Melroy Gateway,Trevithick Port,Leeuwenhoek Hub,van Vogt Camp,Teng Terminal,Komarov City,Barratt Hub,Nicollet Landing"
+ },
+ {
+ "name": "Lalande 22954",
+ "pos_x": 42.8125,
+ "pos_y": 56.96875,
+ "pos_z": 15.21875,
+ "stations": "Nolan Orbital,Kotzebue Port,Springer Settlement"
+ },
+ {
+ "name": "Lalande 2450",
+ "pos_x": -44.21875,
+ "pos_y": -57.59375,
+ "pos_z": -42.46875,
+ "stations": "Czerny Terminal,Acton Hub,Wyeth City"
+ },
+ {
+ "name": "Lalande 26630",
+ "pos_x": 26.53125,
+ "pos_y": 70.8125,
+ "pos_z": 72.75,
+ "stations": "Coelho Depot,Low Colony,Gregory Survey,Thurston Dock"
+ },
+ {
+ "name": "Lalande 2682",
+ "pos_x": -41.875,
+ "pos_y": -49.71875,
+ "pos_z": -42.125,
+ "stations": "Slusser Colony"
+ },
+ {
+ "name": "Lalande 27055",
+ "pos_x": -14.75,
+ "pos_y": 52.5625,
+ "pos_z": 23.03125,
+ "stations": "Lunan Palace,d'Eyncourt Gateway,Ostrander Orbital,RenenBellot Terminal,Leiber Gateway,Griffith Landing,Narvaez Horizons,Robinson Terminal,Sheckley Terminal,Polya Terminal,Rothfuss' Inheritance"
+ },
+ {
+ "name": "Lalande 27958",
+ "pos_x": -38,
+ "pos_y": 91.6875,
+ "pos_z": 47.21875,
+ "stations": "Kirchoff Port,Baydukov Arsenal,Clayton Arena"
+ },
+ {
+ "name": "Lalande 28607",
+ "pos_x": 9.0625,
+ "pos_y": 103.5625,
+ "pos_z": 159.09375,
+ "stations": "Chern Ring,Lee Terminal,Huberath Horizons,Vasquez de Coronado Terminal"
+ },
+ {
+ "name": "Lalande 2933",
+ "pos_x": -112.46875,
+ "pos_y": 7.6875,
+ "pos_z": -88.8125,
+ "stations": "Moffitt Point,Pierres Relay"
+ },
+ {
+ "name": "Lalande 29437",
+ "pos_x": -23.21875,
+ "pos_y": 57.03125,
+ "pos_z": 68.78125,
+ "stations": "Tesla Settlement,Remec Base"
+ },
+ {
+ "name": "Lalande 29917",
+ "pos_x": -26.53125,
+ "pos_y": 22.15625,
+ "pos_z": -4.5625,
+ "stations": "Parsons Platform,Shepard Port,Brust Vision"
+ },
+ {
+ "name": "Lalande 30044",
+ "pos_x": -39.375,
+ "pos_y": 74.78125,
+ "pos_z": 110.75,
+ "stations": "Musabayev Survey,Turtledove Enterprise,Aleksandrov Enterprise,Underwood Holdings"
+ },
+ {
+ "name": "Lalande 30271",
+ "pos_x": -75.4375,
+ "pos_y": 83.03125,
+ "pos_z": 59.9375,
+ "stations": "Gerrold Lab,Williams Settlement,Panshin Terminal,Griffith Silo"
+ },
+ {
+ "name": "Lalande 30699",
+ "pos_x": -64.34375,
+ "pos_y": 48.96875,
+ "pos_z": -10.875,
+ "stations": "Filipchenko Gateway,Walker Horizons,Kazantsev Silo"
+ },
+ {
+ "name": "Lalande 3118",
+ "pos_x": -25.75,
+ "pos_y": 38,
+ "pos_z": 126.6875,
+ "stations": "Witt Orbital,Al-Kashi Hub,MacLeod Arsenal,Block's Folly"
+ },
+ {
+ "name": "Lalande 31528",
+ "pos_x": -76.875,
+ "pos_y": 65.625,
+ "pos_z": 125.90625,
+ "stations": "Derleth Dock"
+ },
+ {
+ "name": "Lalande 31662",
+ "pos_x": -61.53125,
+ "pos_y": 46.875,
+ "pos_z": 56.15625,
+ "stations": "England Ring,Morgan Installation,Land Enterprise,Wilcutt City"
+ },
+ {
+ "name": "Lalande 32151",
+ "pos_x": -123.375,
+ "pos_y": 80.4375,
+ "pos_z": -7.21875,
+ "stations": "Ryman Works,Rangarajan Horizons,Lubin Forum,Lee Gateway,Dampier Terminal,Bohnhoff Ring"
+ },
+ {
+ "name": "Lalande 34968",
+ "pos_x": -156.34375,
+ "pos_y": 77.21875,
+ "pos_z": -20.28125,
+ "stations": "Levy Settlement,Bixby Colony,Russell Relay"
+ },
+ {
+ "name": "Lalande 35998",
+ "pos_x": -143.3125,
+ "pos_y": 31.5,
+ "pos_z": 63.09375,
+ "stations": "Tarelkin Works"
+ },
+ {
+ "name": "Lalande 37120",
+ "pos_x": -95.5,
+ "pos_y": 11.3125,
+ "pos_z": 39.90625,
+ "stations": "Poleshchuk Hub,Voss Dock,Tani Enterprise,Kizim Ring,Khayyam Terminal,Zhigang Port,Banks Gateway,Oefelein Dock,Bradshaw Survey,Menzies' Folly,Keyes Enterprise"
+ },
+ {
+ "name": "Lalande 37923",
+ "pos_x": -84.125,
+ "pos_y": 38.9375,
+ "pos_z": -28.15625,
+ "stations": "Wescott Dock,Lambert Refinery,Al Saud Gateway,Kaleri Dock,Shaw Orbital"
+ },
+ {
+ "name": "Lalande 38451",
+ "pos_x": -59.15625,
+ "pos_y": 3.625,
+ "pos_z": 15.65625,
+ "stations": "Savery Plant,Underwood Platform,Reilly Landing,Artsebarsky Vision"
+ },
+ {
+ "name": "Lalande 38626",
+ "pos_x": -116.96875,
+ "pos_y": 22.9375,
+ "pos_z": 4.5,
+ "stations": "Al-Jazari Orbital,Fincke Ring,Barry Hub,Shunn Prospect,Bondar Terminal,Kerwin Dock,Guidoni Barracks,Garriott Observatory"
+ },
+ {
+ "name": "Lalande 39866",
+ "pos_x": -43.875,
+ "pos_y": -22.46875,
+ "pos_z": 35.28125,
+ "stations": "Dzhanibekov Hub,Goddard City,Jernigan Orbital,Yaping City,Land Enterprise,Rontgen Orbital,Tyurin Terminal,Phillips Legacy,Merbold Terminal"
+ },
+ {
+ "name": "Lalande 40728",
+ "pos_x": -132.25,
+ "pos_y": -9.21875,
+ "pos_z": 16.34375,
+ "stations": "Wohler Station,Merbold Orbital,Sekelj Gateway,Mille Works,Humboldt Enterprise"
+ },
+ {
+ "name": "Lalande 4141",
+ "pos_x": -39.65625,
+ "pos_y": -49.59375,
+ "pos_z": -60.96875,
+ "stations": "4A504D,Shinn Base,Cortes Reach"
+ },
+ {
+ "name": "Lalande 42128",
+ "pos_x": -78.28125,
+ "pos_y": -76.21875,
+ "pos_z": 58.28125,
+ "stations": "Searfoss Stop,Lundwall Platform"
+ },
+ {
+ "name": "Lalande 4268",
+ "pos_x": -13.8125,
+ "pos_y": -64.125,
+ "pos_z": -44.84375,
+ "stations": "Auer Base,Stairs Port,Hill Ring,Lowry Ring"
+ },
+ {
+ "name": "Lalande 43492",
+ "pos_x": -79.90625,
+ "pos_y": -58.71875,
+ "pos_z": 20.4375,
+ "stations": "Broderick Reach,Polansky Hub,Burkin Arsenal"
+ },
+ {
+ "name": "Lalande 44318",
+ "pos_x": -119.9375,
+ "pos_y": -26.6875,
+ "pos_z": -20.71875,
+ "stations": "Jemison Point"
+ },
+ {
+ "name": "Lalande 45165",
+ "pos_x": -91.375,
+ "pos_y": -74.90625,
+ "pos_z": -0.21875,
+ "stations": "Burstein Ring,Thurston Co-operative,Morey Landing"
+ },
+ {
+ "name": "Lalande 45292",
+ "pos_x": -51.21875,
+ "pos_y": -76.625,
+ "pos_z": 13.53125,
+ "stations": "Phillips City,Carpini Landing"
+ },
+ {
+ "name": "Lalande 46867",
+ "pos_x": -103.84375,
+ "pos_y": -69.9375,
+ "pos_z": -35.6875,
+ "stations": "Popovich City,Born Ring,Fossey Port"
+ },
+ {
+ "name": "Lalande 5761",
+ "pos_x": -43.875,
+ "pos_y": -58.75,
+ "pos_z": -108.4375,
+ "stations": "Pook Hub,Gessi Vision"
+ },
+ {
+ "name": "Lalande 6320",
+ "pos_x": 5.625,
+ "pos_y": -36.65625,
+ "pos_z": -33.875,
+ "stations": "Stephen Varey's Station,Stephen Young's Academy,Marshall Legacy"
+ },
+ {
+ "name": "Lalande 8441",
+ "pos_x": -69.78125,
+ "pos_y": 36.09375,
+ "pos_z": -70.3125,
+ "stations": "Barreiros Gateway,Fife Hub,Clebsch Enterprise,Pryor Enterprise,Manning Survey,Mourelle Orbital,Bisson City,Godwin Terminal,Hiraga Enterprise,Krylov Terminal,Alexandria Works,Cartwright Base"
+ },
+ {
+ "name": "Lalande 9428",
+ "pos_x": -69.3125,
+ "pos_y": 33.625,
+ "pos_z": -104.59375,
+ "stations": "Jendrassik Terminal,Magnus Orbital,Gell-Mann Colony,Pausch Refinery,Lee Works"
+ },
+ {
+ "name": "Lalande 9828",
+ "pos_x": -45.125,
+ "pos_y": 22.625,
+ "pos_z": -85.53125,
+ "stations": "Adams Orbital,Bayliss Bastion,Schweinfurth Survey"
+ },
+ {
+ "name": "Lalumaskab",
+ "pos_x": -100.125,
+ "pos_y": 5.96875,
+ "pos_z": -127.9375,
+ "stations": "Soto Orbital,McHugh Terminal,Griffin Terminal,Saavedra Ring"
+ },
+ {
+ "name": "Lalurusat",
+ "pos_x": 9.625,
+ "pos_y": 78.40625,
+ "pos_z": 137.53125,
+ "stations": "Darlton Port,Holden Vision"
+ },
+ {
+ "name": "Laluwang",
+ "pos_x": 159.1875,
+ "pos_y": -100.21875,
+ "pos_z": 58.5,
+ "stations": "Aller Mines,Patry Survey,Plaskett Oasis,Eckford's Inheritance"
+ },
+ {
+ "name": "Lamaku",
+ "pos_x": -25.28125,
+ "pos_y": -49.125,
+ "pos_z": 24.65625,
+ "stations": "Northrop City,Fulton Works,Hornblower City,Yurchikhin Port"
+ },
+ {
+ "name": "Lambaana",
+ "pos_x": 68.5,
+ "pos_y": -113.625,
+ "pos_z": -123.875,
+ "stations": "Kagawa Works"
+ },
+ {
+ "name": "Lambayeque",
+ "pos_x": 120.53125,
+ "pos_y": -104.75,
+ "pos_z": -9.34375,
+ "stations": "Beriev Mines,d'Arrest Beacon"
+ },
+ {
+ "name": "Lambda Andromedae",
+ "pos_x": -78.21875,
+ "pos_y": -21.40625,
+ "pos_z": -29.09375,
+ "stations": "Nourse Orbital,Morris Barracks,Franklin Port,Bounds Horizons,Moore Lab,Conway City,Flynn Horizons,Narbeth Horizons,Fidalgo Vision"
+ },
+ {
+ "name": "Lambda Arae",
+ "pos_x": 21.03125,
+ "pos_y": -12.5625,
+ "pos_z": 65.5,
+ "stations": "Onnes Station,Gagnan City,Wiberg Orbital,Walker Orbital,Fife Vision,Fraley Station,Larbalestier Legacy,Litke Landing,Shepherd Settlement,Panshin Installation,Gibson Barracks,Redi Market,Laplace Landing"
+ },
+ {
+ "name": "Lambda Horologii",
+ "pos_x": 93.6875,
+ "pos_y": -129.125,
+ "pos_z": 22.1875,
+ "stations": "Klaukkala City,Arp Terminal"
+ },
+ {
+ "name": "Lambda Hydri",
+ "pos_x": 130.9375,
+ "pos_y": -143.03125,
+ "pos_z": 85.15625,
+ "stations": "Hansteen Depot"
+ },
+ {
+ "name": "Lambda Muscae",
+ "pos_x": 113.09375,
+ "pos_y": -10.875,
+ "pos_z": 57.0625,
+ "stations": "Grzimek Dock,Tuan Holdings"
+ },
+ {
+ "name": "Lambda-1 Tucanae",
+ "pos_x": 116.65625,
+ "pos_y": -153.15625,
+ "pos_z": 74.46875,
+ "stations": "Riess Hub,Jones Dock,Pimi Orbital,Bainbridge Orbital,Harawi Terminal,Malvern Orbital,Longomontanus Terminal,Riess Station,Mohun Forum,Antonov Terminal,Neff's Claim"
+ },
+ {
+ "name": "Lambda-2 Fornacis",
+ "pos_x": 28.8125,
+ "pos_y": -76.75,
+ "pos_z": -18.75,
+ "stations": "Kepler Hangar,Wellman Settlement"
+ },
+ {
+ "name": "Lambda-2 Phoenicis",
+ "pos_x": 31.15625,
+ "pos_y": -106.21875,
+ "pos_z": 25.46875,
+ "stations": "Sukhoi Terminal,Izumikawa Orbital,Stevenson Vision,Kobayashi Dock,Zel'dovich Ring,Struzan Vision,Elmore Camp,Meyrink Vision,Peltier Vision,Kerimov Keep,Schumacher Station"
+ },
+ {
+ "name": "Lambda-2 Tucanae",
+ "pos_x": 125.9375,
+ "pos_y": -164.5625,
+ "pos_z": 79.34375,
+ "stations": "McQuarrie Landing,Daqing Terminal,Farghani Landing"
+ },
+ {
+ "name": "Lambi",
+ "pos_x": 95.34375,
+ "pos_y": 9.5625,
+ "pos_z": -30.09375,
+ "stations": "Maine Barracks,Barnes Landing"
+ },
+ {
+ "name": "Lambo",
+ "pos_x": 115.375,
+ "pos_y": -82.3125,
+ "pos_z": 110.59375,
+ "stations": "Common Terminal,Lasswitz's Inheritance,Boscovich Landing,Hariot Prospect,Abasheli Palace"
+ },
+ {
+ "name": "Lambog",
+ "pos_x": 167.53125,
+ "pos_y": 2.3125,
+ "pos_z": -40.71875,
+ "stations": "Bryant Dock,McDevitt Installation,Bass Survey,Williams Mines"
+ },
+ {
+ "name": "Lambur",
+ "pos_x": -69.46875,
+ "pos_y": 29.1875,
+ "pos_z": 146.65625,
+ "stations": "Bean Survey,Feoktistov Relay,Fozard Observatory"
+ },
+ {
+ "name": "Lamgana",
+ "pos_x": 24.9375,
+ "pos_y": -9.78125,
+ "pos_z": -147.375,
+ "stations": "Semeonis Dock,Monge Mines,Williamson Dock,Harris Survey,Gottlob Frege Penal colony"
+ },
+ {
+ "name": "Lamgang",
+ "pos_x": -122.21875,
+ "pos_y": -5.9375,
+ "pos_z": -34.15625,
+ "stations": "MacLeod Platform"
+ },
+ {
+ "name": "Lamgani",
+ "pos_x": 74.75,
+ "pos_y": -202.78125,
+ "pos_z": -33.65625,
+ "stations": "Lilienthal Platform,Adelman Station,Hassanein Settlement,Ashbrook Orbital,Tennyson d'Eyncourt Prospect,Vancouver Keep"
+ },
+ {
+ "name": "Lamia",
+ "pos_x": -105.75,
+ "pos_y": -14.46875,
+ "pos_z": 42.4375,
+ "stations": "Noon Port,Bertin Survey"
+ },
+ {
+ "name": "Lampade",
+ "pos_x": 28.28125,
+ "pos_y": -98.09375,
+ "pos_z": 46.9375,
+ "stations": "Encke Gateway,Charlois Dock,Ashman Dock,Walters Penal colony,Niemeyer Enterprise,Mechain Ring"
+ },
+ {
+ "name": "Lan Caihe",
+ "pos_x": -7.15625,
+ "pos_y": 16,
+ "pos_z": -119.34375,
+ "stations": "Zillig Dock,Nachtigal City,Stasheff Arena"
+ },
+ {
+ "name": "Lan Disk",
+ "pos_x": -41.5625,
+ "pos_y": -90.96875,
+ "pos_z": -21.5,
+ "stations": "Grimwood Hub,Fourier Port,Garcia Station,Tall Orbital,Cadamosto Arsenal,Ordway Holdings,Crook Port,Farkas Hub"
+ },
+ {
+ "name": "Lan Gundi",
+ "pos_x": -49.125,
+ "pos_y": -79,
+ "pos_z": -70.375,
+ "stations": "Pohl Refinery,Moisuc Orbital,Collins Bastion,Verrazzano Base,Cernan Escape"
+ },
+ {
+ "name": "Lan Samba",
+ "pos_x": -126.125,
+ "pos_y": -43.65625,
+ "pos_z": 69.25,
+ "stations": "Meyrink Settlement,Cook Base"
+ },
+ {
+ "name": "Lan Tie Yu",
+ "pos_x": -33.34375,
+ "pos_y": 98.40625,
+ "pos_z": 86.75,
+ "stations": "Herrington Vision,Nowak Holdings,Appel Terminal,Baker Penal colony"
+ },
+ {
+ "name": "Lan Tzak",
+ "pos_x": -104.15625,
+ "pos_y": 2.1875,
+ "pos_z": 41.0625,
+ "stations": "Jacobi Platform,Hamilton Holdings,Reynolds Landing,Luiken Bastion,Palmer Beacon"
+ },
+ {
+ "name": "Lan Wang",
+ "pos_x": 7.59375,
+ "pos_y": -44.75,
+ "pos_z": 37.03125,
+ "stations": "Fung Orbital,Teng-hui Ring,Hoffman Enterprise"
+ },
+ {
+ "name": "Lan Yu",
+ "pos_x": 179.90625,
+ "pos_y": -116.21875,
+ "pos_z": -9.46875,
+ "stations": "Bohme Stop"
+ },
+ {
+ "name": "Lanciansene",
+ "pos_x": 125.09375,
+ "pos_y": 0.25,
+ "pos_z": -60.6875,
+ "stations": "McDonald Relay,Gibson Ring,O'Leary Vision"
+ },
+ {
+ "name": "Landji",
+ "pos_x": 141.09375,
+ "pos_y": 39.0625,
+ "pos_z": 15.6875,
+ "stations": "Gernsback Landing,Coulomb Depot"
+ },
+ {
+ "name": "Landuma",
+ "pos_x": 65.5625,
+ "pos_y": -173.4375,
+ "pos_z": 20.1875,
+ "stations": "Wachmann Terminal,Berkey Hub,Nelson Base"
+ },
+ {
+ "name": "Lang",
+ "pos_x": 41.3125,
+ "pos_y": -205.75,
+ "pos_z": 95.84375,
+ "stations": "Couper Outpost,McCrea Point"
+ },
+ {
+ "name": "Lang Yupi",
+ "pos_x": -43.0625,
+ "pos_y": -97.21875,
+ "pos_z": 80.875,
+ "stations": "Young Point,Walters Camp"
+ },
+ {
+ "name": "Langjela",
+ "pos_x": 120.96875,
+ "pos_y": -59.1875,
+ "pos_z": -20.15625,
+ "stations": "Truman Landing,Brule Holdings,Shirazi Port"
+ },
+ {
+ "name": "Langkang",
+ "pos_x": 159.03125,
+ "pos_y": -23.34375,
+ "pos_z": 21.84375,
+ "stations": "Pilcher Settlement,i Sola Platform"
+ },
+ {
+ "name": "Langloines",
+ "pos_x": -4.46875,
+ "pos_y": -189.5625,
+ "pos_z": 41.34375,
+ "stations": "Rigaux Vision"
+ },
+ {
+ "name": "Langoromes",
+ "pos_x": 117.125,
+ "pos_y": -82.28125,
+ "pos_z": 100.875,
+ "stations": "Maughmer Depot,Boss Enterprise,Froud Relay"
+ },
+ {
+ "name": "Langu",
+ "pos_x": 49.84375,
+ "pos_y": -66.21875,
+ "pos_z": -10,
+ "stations": "Maitz Platform,Parker Installation,Alfven Terminal,Jeschke Penal colony"
+ },
+ {
+ "name": "Lani",
+ "pos_x": 1.125,
+ "pos_y": -107.53125,
+ "pos_z": -31.53125,
+ "stations": "Swanson Station,Thorne Enterprise,al-Din Depot,Carson Station"
+ },
+ {
+ "name": "Lansbury",
+ "pos_x": 91.4375,
+ "pos_y": 63.625,
+ "pos_z": 36.125,
+ "stations": "Ivens Landing,Landis Settlement,Henson Laboratory,Thomson Orbital"
+ },
+ {
+ "name": "Lao Gobe",
+ "pos_x": -100.90625,
+ "pos_y": -32,
+ "pos_z": 12.0625,
+ "stations": "Rodrigues Terminal"
+ },
+ {
+ "name": "Lao Gobed",
+ "pos_x": 75.90625,
+ "pos_y": 25.21875,
+ "pos_z": 125.8125,
+ "stations": "Jendrassik Dock,Hobaugh City"
+ },
+ {
+ "name": "Lao Jun",
+ "pos_x": -25.40625,
+ "pos_y": -64.25,
+ "pos_z": 137.78125,
+ "stations": "Szilard Hub,Wells Escape,Schroeder Silo"
+ },
+ {
+ "name": "Lao Wangu",
+ "pos_x": -52.71875,
+ "pos_y": -118.0625,
+ "pos_z": 81.84375,
+ "stations": "Curbeam Mine,Dann Horizons"
+ },
+ {
+ "name": "Lao Yanes",
+ "pos_x": 127.15625,
+ "pos_y": -115,
+ "pos_z": 59.40625,
+ "stations": "Proctor Vision,Milne Survey,Orbik Port"
+ },
+ {
+ "name": "Lao Yano",
+ "pos_x": -74.75,
+ "pos_y": 72.40625,
+ "pos_z": -89.71875,
+ "stations": "Moore Station,Serling Works,Brin Enterprise,Bentham Vision"
+ },
+ {
+ "name": "Lao Zi",
+ "pos_x": -7.4375,
+ "pos_y": -153.46875,
+ "pos_z": 37.0625,
+ "stations": "Shepherd Orbital,Navigator Orbital,Hennepin Dock,Rand Terminal,van Vogt City,Danvers Dock,Wafer City,Sutcliffe Station,Ivens Works,Niven's Progress,Lander Legacy,Davis Legacy"
+ },
+ {
+ "name": "Lapannodaya",
+ "pos_x": 77.125,
+ "pos_y": 27.09375,
+ "pos_z": 32.75,
+ "stations": "Zhen Colony"
+ },
+ {
+ "name": "Larais",
+ "pos_x": 65.96875,
+ "pos_y": 43.25,
+ "pos_z": 51.5,
+ "stations": "Volta Landing,Morris Base,Burbank Orbital"
+ },
+ {
+ "name": "Larda",
+ "pos_x": -1.1875,
+ "pos_y": -2.6875,
+ "pos_z": -160.59375,
+ "stations": "Powers Horizons,Dias Observatory,Wyndham Installation,Maine Mines,Foden Ring"
+ },
+ {
+ "name": "Lardhampt",
+ "pos_x": 18.0625,
+ "pos_y": 59.78125,
+ "pos_z": 57.1875,
+ "stations": "Buckey Terminal,Gresley Terminal,Herbert Silo"
+ },
+ {
+ "name": "Larg",
+ "pos_x": 152.5625,
+ "pos_y": -138.46875,
+ "pos_z": 132.9375,
+ "stations": "Kagawa Survey,Porco Holdings,Struve Colony,Boyer Prospect,Balog's Inheritance"
+ },
+ {
+ "name": "Largam",
+ "pos_x": -62.875,
+ "pos_y": -120.875,
+ "pos_z": -16.71875,
+ "stations": "Napier Terminal,Forsskal Hub"
+ },
+ {
+ "name": "Largarayu",
+ "pos_x": 9.65625,
+ "pos_y": 31.53125,
+ "pos_z": 81.34375,
+ "stations": "Mills Enterprise,Wilcutt Refinery,Chilton Port,Voss Terminal,Rond d'Alembert Vision"
+ },
+ {
+ "name": "Larri",
+ "pos_x": -103.125,
+ "pos_y": 78.34375,
+ "pos_z": 99.25,
+ "stations": "Isherwood Dock,Sarrantonio Colony,Perga Landing"
+ },
+ {
+ "name": "Larrid",
+ "pos_x": 65.625,
+ "pos_y": 45.6875,
+ "pos_z": 102.875,
+ "stations": "Rae Terminal,Shipton Base"
+ },
+ {
+ "name": "Las Vegaria",
+ "pos_x": 69.6875,
+ "pos_y": -216.28125,
+ "pos_z": 15.34375,
+ "stations": "i Sola Terminal"
+ },
+ {
+ "name": "Las Velini",
+ "pos_x": 143,
+ "pos_y": 6.59375,
+ "pos_z": -7.65625,
+ "stations": "Dyr Retreat,Bailey Settlement,Kessel Bastion"
+ },
+ {
+ "name": "Lati",
+ "pos_x": 16.28125,
+ "pos_y": 31.96875,
+ "pos_z": -91.65625,
+ "stations": "Hoyle Base,Watts Settlement,Quaglia Camp"
+ },
+ {
+ "name": "Latjal",
+ "pos_x": -26.6875,
+ "pos_y": 55.34375,
+ "pos_z": 80.78125,
+ "stations": "Skvortsov Terminal,Watt Terminal,Barbaro Relay,Ashby Terminal"
+ },
+ {
+ "name": "Latjang",
+ "pos_x": -64.78125,
+ "pos_y": 21.25,
+ "pos_z": 136.4375,
+ "stations": "Currie Holdings,Merchiston Base"
+ },
+ {
+ "name": "Latji",
+ "pos_x": 98.25,
+ "pos_y": 0.0625,
+ "pos_z": 103.34375,
+ "stations": "al-Din Dock"
+ },
+ {
+ "name": "Latjil",
+ "pos_x": -41.59375,
+ "pos_y": -116.03125,
+ "pos_z": -19.625,
+ "stations": "Al-Farabi Hub,Potter Enterprise,Payton Landing,Thorne Silo,Nicollet Base"
+ },
+ {
+ "name": "Latobici",
+ "pos_x": 92.9375,
+ "pos_y": -12.71875,
+ "pos_z": 54,
+ "stations": "Schiltberger City,Nagata Depot,Fiennes Platform"
+ },
+ {
+ "name": "Latobrigi",
+ "pos_x": 169.125,
+ "pos_y": 21.875,
+ "pos_z": -35.6875,
+ "stations": "Blair Orbital,Sargent Legacy,Koontz Settlement"
+ },
+ {
+ "name": "Latola",
+ "pos_x": 28.34375,
+ "pos_y": 131.625,
+ "pos_z": 69.75,
+ "stations": "Wait Colburn Gateway"
+ },
+ {
+ "name": "Latones",
+ "pos_x": 22.03125,
+ "pos_y": -24.21875,
+ "pos_z": 152.03125,
+ "stations": "Clark Ring,Titov Survey,Henize Hub"
+ },
+ {
+ "name": "Latoras",
+ "pos_x": -91.96875,
+ "pos_y": 85.78125,
+ "pos_z": -12.53125,
+ "stations": "Bates Vision,Kessel Colony,Nearchus Vision"
+ },
+ {
+ "name": "Latorioson",
+ "pos_x": 17.28125,
+ "pos_y": -69.15625,
+ "pos_z": -107.53125,
+ "stations": "Lysenko City,Bowersox Terminal,Cleave Landing"
+ },
+ {
+ "name": "Latormodur",
+ "pos_x": 64.6875,
+ "pos_y": -133.5,
+ "pos_z": -61.65625,
+ "stations": "Jung Terminal,van de Hulst Enterprise,Taylor Enterprise,Rutan Station"
+ },
+ {
+ "name": "Latu",
+ "pos_x": -109.375,
+ "pos_y": 31.40625,
+ "pos_z": 48.625,
+ "stations": "Conway Works,Midgeley Settlement,Rae Camp,Eisele Landing"
+ },
+ {
+ "name": "Latuba",
+ "pos_x": 140.03125,
+ "pos_y": -71.4375,
+ "pos_z": 4.96875,
+ "stations": "Soukup City,Doctorow Holdings"
+ },
+ {
+ "name": "Latuca",
+ "pos_x": -99.8125,
+ "pos_y": -19.5625,
+ "pos_z": -99.4375,
+ "stations": "Le Guin Mines"
+ },
+ {
+ "name": "Latucan",
+ "pos_x": -92.53125,
+ "pos_y": -137.65625,
+ "pos_z": 12.6875,
+ "stations": "Wilhelm Orbital"
+ },
+ {
+ "name": "Latucano",
+ "pos_x": -88.84375,
+ "pos_y": 85.0625,
+ "pos_z": 73.84375,
+ "stations": "Jeury Hub,Ore Base"
+ },
+ {
+ "name": "Latugag",
+ "pos_x": 149.25,
+ "pos_y": -102.46875,
+ "pos_z": 73.53125,
+ "stations": "Darwin Reformatory"
+ },
+ {
+ "name": "Latugara",
+ "pos_x": -110.53125,
+ "pos_y": -18.8125,
+ "pos_z": 104.625,
+ "stations": "Love Orbital,Czerny Dock,Franke Base,Helmholtz Enterprise"
+ },
+ {
+ "name": "Latunde",
+ "pos_x": 172.84375,
+ "pos_y": -153.46875,
+ "pos_z": -39.28125,
+ "stations": "Henry Prospect,Schwarzschild Platform,Crown Point"
+ },
+ {
+ "name": "Latured",
+ "pos_x": 76.53125,
+ "pos_y": 10.875,
+ "pos_z": 123.0625,
+ "stations": "Hoyle Relay,Bosch Retreat"
+ },
+ {
+ "name": "Laturesingo",
+ "pos_x": 162.75,
+ "pos_y": -70.84375,
+ "pos_z": 63.59375,
+ "stations": "Fedden Orbital,Barnes Terminal,Houten-Groeneveld Vision"
+ },
+ {
+ "name": "Latuslogii",
+ "pos_x": -99.90625,
+ "pos_y": -162.40625,
+ "pos_z": -52.9375,
+ "stations": "King Landing,Beekman Beacon,McKee Vision"
+ },
+ {
+ "name": "Latuveanes",
+ "pos_x": 113.65625,
+ "pos_y": -11.625,
+ "pos_z": 56.09375,
+ "stations": "Hyecho Vision,Kazantsev City,Elvstrom Terminal"
+ },
+ {
+ "name": "Laukaitu",
+ "pos_x": 55.25,
+ "pos_y": -117.875,
+ "pos_z": -83.9375,
+ "stations": "Makarov Depot,Cummings Prospect,Lee Horizons"
+ },
+ {
+ "name": "Laukama",
+ "pos_x": -139.1875,
+ "pos_y": 88.53125,
+ "pos_z": -28.03125,
+ "stations": "Plexico Settlement,Paulo da Gama Vision,Detmer Lab"
+ },
+ {
+ "name": "Laukan",
+ "pos_x": 89.5,
+ "pos_y": -47.15625,
+ "pos_z": 105.71875,
+ "stations": "Apianus Station,Nakamura Gateway,Mandel Mines,Ziegler Enterprise,Tanner Depot,Heinkel Vision"
+ },
+ {
+ "name": "Laukang",
+ "pos_x": -3.28125,
+ "pos_y": 95.875,
+ "pos_z": 75.1875,
+ "stations": "Matheson Orbital"
+ },
+ {
+ "name": "Laukas",
+ "pos_x": -72.75,
+ "pos_y": -96.6875,
+ "pos_z": -81.53125,
+ "stations": "Barbosa Platform"
+ },
+ {
+ "name": "Laukates",
+ "pos_x": 14.59375,
+ "pos_y": 88.25,
+ "pos_z": 74.40625,
+ "stations": "Coulomb Plant,Kuhn Platform"
+ },
+ {
+ "name": "Laukese",
+ "pos_x": 104.78125,
+ "pos_y": -24.09375,
+ "pos_z": 65.78125,
+ "stations": "Moran Landing,Loncke Survey,Dillon Dock,Giunta Gateway,Oren Vision,Hawkes Settlement,Walter City,Henry Vision,Angstrom City,Seitter Orbital,Antoniadi Vision,Neumann Gateway,Gehrels Station,Neujmin Depot,Thesiger's Inheritance,Alvares Lab"
+ },
+ {
+ "name": "Lauket",
+ "pos_x": 5.5,
+ "pos_y": -142.40625,
+ "pos_z": -70.1875,
+ "stations": "Lander Retreat,Carter Horizons"
+ },
+ {
+ "name": "Laukh",
+ "pos_x": -93.6875,
+ "pos_y": -92.03125,
+ "pos_z": -132.34375,
+ "stations": "Staden Dock,Shirley Prospect,Walker Reach"
+ },
+ {
+ "name": "Lauksa",
+ "pos_x": 17.8125,
+ "pos_y": -33.96875,
+ "pos_z": 60.6875,
+ "stations": "Smith Enterprise,Teller Ring,Park Beacon,Williams Silo"
+ },
+ {
+ "name": "Lauma",
+ "pos_x": 16.8125,
+ "pos_y": -43.8125,
+ "pos_z": 38.59375,
+ "stations": "Pribylov Relay,Vian Colony,Foda Port,Afanasyev Dock"
+ },
+ {
+ "name": "Laumaia",
+ "pos_x": 83.25,
+ "pos_y": -152.21875,
+ "pos_z": 5.375,
+ "stations": "Vess Orbital,Hiyya Depot,Hirayama Enterprise,Bohme Platform"
+ },
+ {
+ "name": "Laumas",
+ "pos_x": 132.125,
+ "pos_y": 22.375,
+ "pos_z": -116.875,
+ "stations": "Cady Port,Hoffman Terminal,Vinge Station"
+ },
+ {
+ "name": "Laumerthur",
+ "pos_x": 110,
+ "pos_y": -77.34375,
+ "pos_z": -85.75,
+ "stations": "Yanai Depot"
+ },
+ {
+ "name": "Laur",
+ "pos_x": 89.53125,
+ "pos_y": -113.65625,
+ "pos_z": -23.34375,
+ "stations": "Alexandria Ring,Wigura Gateway,Theodorsen Terminal,De Lay Station,Flettner Dock,Leuschner Station,Islam Plant,Hill Terminal,Tiptree Barracks,Roe Orbital,Barlowe Port,McMullen Prospect,Asaro Terminal,Euthymenes Camp"
+ },
+ {
+ "name": "Lauricocha",
+ "pos_x": -59.28125,
+ "pos_y": 140.9375,
+ "pos_z": -43.125,
+ "stations": "Weinbaum Ring,Tevis Enterprise"
+ },
+ {
+ "name": "Lausang",
+ "pos_x": 16.96875,
+ "pos_y": -82.5,
+ "pos_z": -55.15625,
+ "stations": "The Discordian Society,Black Station,Lambert Exchange,Neugebauer Hangar"
+ },
+ {
+ "name": "Lave",
+ "pos_x": 75.75,
+ "pos_y": 48.75,
+ "pos_z": 70.75,
+ "stations": "Lave Station,Warinus,Castellan Station"
+ },
+ {
+ "name": "LAWD 13",
+ "pos_x": 52.1875,
+ "pos_y": -52,
+ "pos_z": 18.0625,
+ "stations": "Potrykus Platform,Chamitoff City,Muhammad Ibn Battuta Camp"
+ },
+ {
+ "name": "LAWD 17",
+ "pos_x": -1.84375,
+ "pos_y": 39.78125,
+ "pos_z": -39.6875,
+ "stations": "Arkwright Landing,Armstrong Hangar"
+ },
+ {
+ "name": "LAWD 26",
+ "pos_x": 20.90625,
+ "pos_y": -7.5,
+ "pos_z": 3.75,
+ "stations": "Stone's Legacy"
+ },
+ {
+ "name": "LAWD 27",
+ "pos_x": 71.3125,
+ "pos_y": -22.90625,
+ "pos_z": 11.8125,
+ "stations": "Lawson Dock,Malyshev Orbital"
+ },
+ {
+ "name": "LAWD 52",
+ "pos_x": -52.28125,
+ "pos_y": 61.8125,
+ "pos_z": -26.5625,
+ "stations": "Coles Gateway,Leichhardt Enterprise,Tem Terminal,Chorel Arsenal"
+ },
+ {
+ "name": "LAWD 7",
+ "pos_x": -16.4375,
+ "pos_y": -73.03125,
+ "pos_z": -12.34375,
+ "stations": "Burbank Plant,Hedley Dock"
+ },
+ {
+ "name": "LAWD 8",
+ "pos_x": -26.25,
+ "pos_y": -36.9375,
+ "pos_z": -23.75,
+ "stations": "Priestley Colony,Tognini Hub"
+ },
+ {
+ "name": "LAWD 80",
+ "pos_x": -17.59375,
+ "pos_y": -24.78125,
+ "pos_z": 46.1875,
+ "stations": "Cavalieri Station,Haldeman II City,Gamow Prospect"
+ },
+ {
+ "name": "LAWD 96",
+ "pos_x": 3.875,
+ "pos_y": -24.09375,
+ "pos_z": 7.25,
+ "stations": "Pythagoras Vision,Wiener Prospect,Holden Dock,Landsteiner Relay"
+ },
+ {
+ "name": "Lazdones",
+ "pos_x": -70.875,
+ "pos_y": -2.46875,
+ "pos_z": 45.75,
+ "stations": "Gregory Hub,Halsell Hub,Wul Survey,Armstrong Station,Tucker Prospect,Cori Hub,Mendel Station,McCoy Terminal,Crown Terminal,Holdstock Vista"
+ },
+ {
+ "name": "Lazdongand",
+ "pos_x": 142.75,
+ "pos_y": -3.03125,
+ "pos_z": 62.28125,
+ "stations": "Weierstrass Terminal,Borlaug Installation,Garneau Dock"
+ },
+ {
+ "name": "LB 3303",
+ "pos_x": 22.9375,
+ "pos_y": -22.9375,
+ "pos_z": 6.5625,
+ "stations": "Al-Khalili Port,Poindexter Horizons,Haignere Arsenal,Marshall City"
+ },
+ {
+ "name": "LDS 2314",
+ "pos_x": -16.5,
+ "pos_y": 44.5625,
+ "pos_z": -31.875,
+ "stations": "Dobrovolskiy Orbital,Adamson Forum,Ellis Orbital,Shull Station,Thompson Enterprise,McArthur Exchange"
+ },
+ {
+ "name": "LDS 3768",
+ "pos_x": 27.71875,
+ "pos_y": 25.09375,
+ "pos_z": -56.75,
+ "stations": "Gaspar de Lemos Dock,Serre Dock,Fernandes de Queiros Dock"
+ },
+ {
+ "name": "LDS 413",
+ "pos_x": 53.65625,
+ "pos_y": 32.40625,
+ "pos_z": 30.84375,
+ "stations": "Gemar Terminal,Ockels Relay,Friesner Installation,Borlaug Hub"
+ },
+ {
+ "name": "LDS 883",
+ "pos_x": -28.71875,
+ "pos_y": -35.71875,
+ "pos_z": -61.4375,
+ "stations": "Smith Reserve,Barnaby Estate,Tudela Relay,Rae Terminal,Makarov Enterprise"
+ },
+ {
+ "name": "Le Ejja",
+ "pos_x": -112.46875,
+ "pos_y": 36.96875,
+ "pos_z": 22.59375,
+ "stations": "Lasswitz Sanctuary,Shaw Reach"
+ },
+ {
+ "name": "Leerham",
+ "pos_x": -13.625,
+ "pos_y": 44.40625,
+ "pos_z": 76.96875,
+ "stations": "Low Enterprise,Minkowski Depot,Weil Enterprise"
+ },
+ {
+ "name": "Leesti",
+ "pos_x": 72.75,
+ "pos_y": 48.75,
+ "pos_z": 68.25,
+ "stations": "Vatermann LLC,George Lucas,Kolmogorov Hub"
+ },
+ {
+ "name": "Lega",
+ "pos_x": 156.03125,
+ "pos_y": -16.59375,
+ "pos_z": 70.59375,
+ "stations": "Hand Orbital,Remec Orbital,Johnson Vision,J. G. Ballard Base"
+ },
+ {
+ "name": "Legba",
+ "pos_x": 173.125,
+ "pos_y": -128.4375,
+ "pos_z": -35.46875,
+ "stations": "Lalande Port,Bullialdus Holdings"
+ },
+ {
+ "name": "Legees",
+ "pos_x": 73.6875,
+ "pos_y": 67.09375,
+ "pos_z": 69.40625,
+ "stations": "Szilard Gateway,Stefanyshyn-Piper City,Matthews Installation"
+ },
+ {
+ "name": "LEHPM 1902",
+ "pos_x": 20.90625,
+ "pos_y": -100.28125,
+ "pos_z": -13.34375,
+ "stations": "Lomonosov Hub,Nomen Vision,Puleston Penal colony"
+ },
+ {
+ "name": "Lei Bei",
+ "pos_x": 110.78125,
+ "pos_y": 19.34375,
+ "pos_z": -58.84375,
+ "stations": "Sinclair Port,Walz Survey,Wilder's Inheritance"
+ },
+ {
+ "name": "Lei Cherna",
+ "pos_x": -16.25,
+ "pos_y": -109,
+ "pos_z": -89.53125,
+ "stations": "Baffin Refinery,Mukai Dock,Wegener Beacon"
+ },
+ {
+ "name": "Lei Ching",
+ "pos_x": -24.96875,
+ "pos_y": -162.875,
+ "pos_z": 0.875,
+ "stations": "Cartier Refinery,Baliunas Platform,Herbert Prospect,Biruni Beacon"
+ },
+ {
+ "name": "Lei Hai Te",
+ "pos_x": 40.875,
+ "pos_y": -79.28125,
+ "pos_z": 142.4375,
+ "stations": "Vinge Base,Peebles Reach"
+ },
+ {
+ "name": "Lei Heile",
+ "pos_x": 159.84375,
+ "pos_y": -189.5,
+ "pos_z": 15.125,
+ "stations": "Espenak Platform,Baade Port,Macomb Depot"
+ },
+ {
+ "name": "Lei Hesang",
+ "pos_x": 2.71875,
+ "pos_y": -102.1875,
+ "pos_z": 28.875,
+ "stations": "Kirk Colony,Werber's Inheritance,Mayer Dock"
+ },
+ {
+ "name": "Lei Hsini",
+ "pos_x": -2.96875,
+ "pos_y": -42.8125,
+ "pos_z": -164.5625,
+ "stations": "Ivanov Mine"
+ },
+ {
+ "name": "Lei Jen Zu",
+ "pos_x": -28.6875,
+ "pos_y": 26.96875,
+ "pos_z": -84.53125,
+ "stations": "Berliner Dock,Kozeyev Gateway,Leonov Hub,Fremion Barracks,Avicenna Gateway,Freud Dock,Brin Laboratory,Murray Dock"
+ },
+ {
+ "name": "Lei Jing",
+ "pos_x": 31.25,
+ "pos_y": 4.46875,
+ "pos_z": -160.875,
+ "stations": "Krylov Platform,Larson Landing,Crown Prospect,Davis Survey"
+ },
+ {
+ "name": "Lei Kax",
+ "pos_x": 122.53125,
+ "pos_y": -78.90625,
+ "pos_z": -46.4375,
+ "stations": "Gurshtein Installation,Kuchner Terminal"
+ },
+ {
+ "name": "Lei Ku",
+ "pos_x": 97.75,
+ "pos_y": -218.875,
+ "pos_z": 35.96875,
+ "stations": "Common Hub,Fokker Terminal"
+ },
+ {
+ "name": "Lei Lin",
+ "pos_x": -99.59375,
+ "pos_y": 96.25,
+ "pos_z": 58.71875,
+ "stations": "Pausch Relay,Celsius Beacon,Budarin Holdings"
+ },
+ {
+ "name": "Lei Manako",
+ "pos_x": -150.375,
+ "pos_y": 30.3125,
+ "pos_z": -13.9375,
+ "stations": "Alexeyev Orbital,Stokes Orbital,Linenger Penal colony"
+ },
+ {
+ "name": "Lei Mati",
+ "pos_x": 41.15625,
+ "pos_y": -80.375,
+ "pos_z": 75.46875,
+ "stations": "Canty Station,Rosenberg Base,Phillips Orbital"
+ },
+ {
+ "name": "Lei Zi",
+ "pos_x": 56.9375,
+ "pos_y": 44.75,
+ "pos_z": 42.6875,
+ "stations": "Barreiros Beacon,Macedo Station,Gunn Depot"
+ },
+ {
+ "name": "Lekagh",
+ "pos_x": 55.40625,
+ "pos_y": 51.125,
+ "pos_z": -103.4375,
+ "stations": "Dunyach's Progress,Garneau Hub,Szilard Landing"
+ },
+ {
+ "name": "Lekan Yu",
+ "pos_x": 106.1875,
+ "pos_y": 46.0625,
+ "pos_z": 108.9375,
+ "stations": "Dall Mine,Gallun Landing"
+ },
+ {
+ "name": "Lelabo",
+ "pos_x": 133.03125,
+ "pos_y": 38.0625,
+ "pos_z": -51.71875,
+ "stations": "Bulgakov Ring,Selberg City,Pashin Dock,Brooks Barracks"
+ },
+ {
+ "name": "Lelae",
+ "pos_x": -149.125,
+ "pos_y": 19.25,
+ "pos_z": -2.15625,
+ "stations": "Armstrong Terminal,Popovich Platform"
+ },
+ {
+ "name": "Lelalakan",
+ "pos_x": -114,
+ "pos_y": 88.46875,
+ "pos_z": -65.21875,
+ "stations": "Eisele Depot,Dann's Claim,Nikitin Vision"
+ },
+ {
+ "name": "Lelalites",
+ "pos_x": -98.625,
+ "pos_y": -141.625,
+ "pos_z": 85.15625,
+ "stations": "Celebi Landing,Stokes Installation,Cortes Platform"
+ },
+ {
+ "name": "Lelao",
+ "pos_x": 162.4375,
+ "pos_y": 24.25,
+ "pos_z": 17.375,
+ "stations": "Bottego Settlement,Gromov Dock,Scithers Beacon"
+ },
+ {
+ "name": "Lelatuba",
+ "pos_x": -20.375,
+ "pos_y": -104.71875,
+ "pos_z": -50.3125,
+ "stations": "Johnson Landing,Abernathy Beacon"
+ },
+ {
+ "name": "Lelatunji",
+ "pos_x": 42.6875,
+ "pos_y": -128.6875,
+ "pos_z": 105.03125,
+ "stations": "Vuia Port"
+ },
+ {
+ "name": "Leleng Musu",
+ "pos_x": -69.15625,
+ "pos_y": -4.6875,
+ "pos_z": -167.78125,
+ "stations": "McKay Mine,Capek Depot,Barnes Retreat"
+ },
+ {
+ "name": "Lemana",
+ "pos_x": -138.15625,
+ "pos_y": -100.5,
+ "pos_z": -19.21875,
+ "stations": "Vardeman Prospect,Morris Settlement,Friesner Base"
+ },
+ {
+ "name": "Lemastshi",
+ "pos_x": 70.09375,
+ "pos_y": 63.71875,
+ "pos_z": 72,
+ "stations": "Maudslay Mines,Lonchakov Dock,Collins Plant"
+ },
+ {
+ "name": "Lemayak",
+ "pos_x": -171.875,
+ "pos_y": -102.5,
+ "pos_z": 33.96875,
+ "stations": "Moon Outpost"
+ },
+ {
+ "name": "Lembava",
+ "pos_x": -43.25,
+ "pos_y": -64.34375,
+ "pos_z": -77.6875,
+ "stations": "Goldstein Port,Morrison Dock,Strzelecki Observatory,Schachner City"
+ },
+ {
+ "name": "Lemoerdhis",
+ "pos_x": -51.625,
+ "pos_y": -44.34375,
+ "pos_z": -49.4375,
+ "stations": "Thornton Depot"
+ },
+ {
+ "name": "Lemoerige",
+ "pos_x": -4.3125,
+ "pos_y": 99.65625,
+ "pos_z": 117.71875,
+ "stations": "Gaffney Port,Thompson Relay"
+ },
+ {
+ "name": "Lemonites",
+ "pos_x": 29.6875,
+ "pos_y": -77.28125,
+ "pos_z": -61.03125,
+ "stations": "Rochon Base,Hulse Station"
+ },
+ {
+ "name": "Lemovi",
+ "pos_x": 99.96875,
+ "pos_y": -47.71875,
+ "pos_z": 8.5,
+ "stations": "Royo Dock,Kidinnu Landing,Murakami Point"
+ },
+ {
+ "name": "Lemowo",
+ "pos_x": 17.9375,
+ "pos_y": 8.53125,
+ "pos_z": 159.78125,
+ "stations": "Chapman Mines"
+ },
+ {
+ "name": "Lenca",
+ "pos_x": -57.8125,
+ "pos_y": -65.28125,
+ "pos_z": 108.03125,
+ "stations": "Yu Relay,Champlain Survey"
+ },
+ {
+ "name": "Lencali",
+ "pos_x": -8.53125,
+ "pos_y": 10.46875,
+ "pos_z": 67.4375,
+ "stations": "Reynolds Ring,Harrison Depot,Herschel City,Libby Dock"
+ },
+ {
+ "name": "Lenchis",
+ "pos_x": 27.8125,
+ "pos_y": -19.09375,
+ "pos_z": -99.34375,
+ "stations": "Lowry Depot,Dietz Gateway,Volterra Platform,Fisk Port"
+ },
+ {
+ "name": "Lendinji",
+ "pos_x": 15.46875,
+ "pos_y": 66.28125,
+ "pos_z": 136.28125,
+ "stations": "Kennicott Dock,Bear Dock,LeConte Colony,Young Survey"
+ },
+ {
+ "name": "Leng Dana",
+ "pos_x": -81.6875,
+ "pos_y": 58.90625,
+ "pos_z": -143.28125,
+ "stations": "Brin Mines"
+ },
+ {
+ "name": "Lengi",
+ "pos_x": 5.71875,
+ "pos_y": -18.90625,
+ "pos_z": 94.375,
+ "stations": "Weiss Keep,Dzhanibekov Installation,Grover Settlement"
+ },
+ {
+ "name": "Lenginang",
+ "pos_x": -43,
+ "pos_y": 95.78125,
+ "pos_z": -130.40625,
+ "stations": "Napier Beacon"
+ },
+ {
+ "name": "Lengthang",
+ "pos_x": 74.96875,
+ "pos_y": -90.78125,
+ "pos_z": -14.15625,
+ "stations": "Nemere's Pride"
+ },
+ {
+ "name": "Lenguna",
+ "pos_x": 87.90625,
+ "pos_y": -34.6875,
+ "pos_z": 67.90625,
+ "stations": "Gantt Colony"
+ },
+ {
+ "name": "Lengur",
+ "pos_x": -71.53125,
+ "pos_y": 7.28125,
+ "pos_z": -139.59375,
+ "stations": "Chilton Station"
+ },
+ {
+ "name": "Lengye",
+ "pos_x": 100.28125,
+ "pos_y": 111.3125,
+ "pos_z": 48.90625,
+ "stations": "Diesel Terminal"
+ },
+ {
+ "name": "Lenore",
+ "pos_x": 19.9375,
+ "pos_y": 87.28125,
+ "pos_z": -0.375,
+ "stations": "MacVicar Enterprise,Gamow Hub,la Cosa Barracks,Garriott Station,Kregel Station"
+ },
+ {
+ "name": "Lentes",
+ "pos_x": -91.25,
+ "pos_y": 19.3125,
+ "pos_z": 36.0625,
+ "stations": "Baudry Platform,Parsons Reach"
+ },
+ {
+ "name": "Lenty",
+ "pos_x": 29.40625,
+ "pos_y": -22.09375,
+ "pos_z": -159.53125,
+ "stations": "Zindell Depot,Crook Stop,Cameron Plant"
+ },
+ {
+ "name": "Lepcha",
+ "pos_x": 95.15625,
+ "pos_y": 108.1875,
+ "pos_z": -84.3125,
+ "stations": "Converse Station,Henderson Relay,Crichton Barracks"
+ },
+ {
+ "name": "Lepchaimyu",
+ "pos_x": 97.53125,
+ "pos_y": -79.3125,
+ "pos_z": 58.6875,
+ "stations": "Parker's Exile"
+ },
+ {
+ "name": "Lepchana",
+ "pos_x": 165.3125,
+ "pos_y": -108.78125,
+ "pos_z": 50.84375,
+ "stations": "Brooks Base,Hansen Orbital,Poyser Vision"
+ },
+ {
+ "name": "Lepchazi",
+ "pos_x": 130.96875,
+ "pos_y": 53.25,
+ "pos_z": -41.75,
+ "stations": "Taylor Dock,Rusch Vision,Abernathy Terminal"
+ },
+ {
+ "name": "Lepontine",
+ "pos_x": 31.71875,
+ "pos_y": 68.34375,
+ "pos_z": 148.625,
+ "stations": "Murray Horizons,Brin Silo,Morris Installation"
+ },
+ {
+ "name": "Lepontu",
+ "pos_x": -109.0625,
+ "pos_y": 29.875,
+ "pos_z": 111.5625,
+ "stations": "Langford Orbital,North Lab,Anthony Vision,Miller Station,Lee Installation"
+ },
+ {
+ "name": "Leschanatya",
+ "pos_x": -134.875,
+ "pos_y": 12.40625,
+ "pos_z": -74.59375,
+ "stations": "Lucretius Mine"
+ },
+ {
+ "name": "Lesche",
+ "pos_x": 131.09375,
+ "pos_y": -135.9375,
+ "pos_z": -17.65625,
+ "stations": "Pajdusakova Platform,Schiaparelli Vision,Carrington Orbital,Helffrich Colony"
+ },
+ {
+ "name": "Lescherty",
+ "pos_x": 40.5,
+ "pos_y": -136.5625,
+ "pos_z": 157.1875,
+ "stations": "Guth Vision,Vaucouleurs Enterprise,Rubin Terminal,Moore Enterprise"
+ },
+ {
+ "name": "Lese",
+ "pos_x": -23.46875,
+ "pos_y": 42.15625,
+ "pos_z": -98.875,
+ "stations": "Chang-Diaz Orbital,Morgan Port,Samokutyayev Enterprise,Diophantus' Inheritance"
+ },
+ {
+ "name": "Lesheima",
+ "pos_x": 81.625,
+ "pos_y": 3.15625,
+ "pos_z": 20,
+ "stations": "Sturckow Orbital,Prunariu Enterprise,Skvortsov Dock"
+ },
+ {
+ "name": "Leshertrug",
+ "pos_x": -34.8125,
+ "pos_y": -102.875,
+ "pos_z": -100.84375,
+ "stations": "Pausch Port,Potter Landing,Burstein Survey,Balmer Landing"
+ },
+ {
+ "name": "Lesnik",
+ "pos_x": 112.40625,
+ "pos_y": -190.71875,
+ "pos_z": 87.25,
+ "stations": "Mandel Relay,Olahus' Inheritance"
+ },
+ {
+ "name": "Lesovices",
+ "pos_x": 111.1875,
+ "pos_y": 53.65625,
+ "pos_z": -134.59375,
+ "stations": "Keyes Platform,Holdstock Terminal,Lasswitz Installation"
+ },
+ {
+ "name": "Lesovik",
+ "pos_x": 110.40625,
+ "pos_y": -132.40625,
+ "pos_z": 7.1875,
+ "stations": "Pittendreigh Survey,Galindo's Inheritance,Wirtanen Landing"
+ },
+ {
+ "name": "Lethe",
+ "pos_x": 67.15625,
+ "pos_y": -43.0625,
+ "pos_z": -18.59375,
+ "stations": "Bhabha's Claim,Obruchev Bastion,Ewald Landing"
+ },
+ {
+ "name": "Leucing",
+ "pos_x": -10.9375,
+ "pos_y": 85.75,
+ "pos_z": 20,
+ "stations": "Grassmann Orbital,Maury Station,Grimwood Ring"
+ },
+ {
+ "name": "Leucos",
+ "pos_x": 98.65625,
+ "pos_y": -3.375,
+ "pos_z": -5.65625,
+ "stations": "Haignere Ring,Morey Enterprise,Savitskaya Hub,Redi Hub"
+ },
+ {
+ "name": "Leucosi",
+ "pos_x": 161.15625,
+ "pos_y": -66.1875,
+ "pos_z": 25.6875,
+ "stations": "Anderson Hangar,Kelly Hub,Barton Plant,Veron Vision,Korolev Vision"
+ },
+ {
+ "name": "Leucosimha",
+ "pos_x": 95.40625,
+ "pos_y": 40.15625,
+ "pos_z": 15.625,
+ "stations": "Scott Station,Cseszneky City,Polyakov Orbital,Bakewell Settlement"
+ },
+ {
+ "name": "Leucosyen",
+ "pos_x": -95.8125,
+ "pos_y": 105.8125,
+ "pos_z": 115.03125,
+ "stations": "Wolfe Vision"
+ },
+ {
+ "name": "Leunii",
+ "pos_x": 127.1875,
+ "pos_y": -172.59375,
+ "pos_z": -102.25,
+ "stations": "Finlay Platform,Ayton Innes Beacon,Borrelly Escape"
+ },
+ {
+ "name": "Lexovit",
+ "pos_x": 152.65625,
+ "pos_y": -98.0625,
+ "pos_z": 99.78125,
+ "stations": "Muller Base,Dominique's Progress"
+ },
+ {
+ "name": "Lexovits",
+ "pos_x": 79.78125,
+ "pos_y": -182.96875,
+ "pos_z": 141.8125,
+ "stations": "Shea Port,Melotte Dock,Wallis Base"
+ },
+ {
+ "name": "Lezaei",
+ "pos_x": 70.4375,
+ "pos_y": 61.6875,
+ "pos_z": -88.125,
+ "stations": "Wolf Vision"
+ },
+ {
+ "name": "LF 8 +16 41",
+ "pos_x": 23.90625,
+ "pos_y": 1.59375,
+ "pos_z": -86.59375,
+ "stations": "Szentmartony Hub,Wright Vision,Gessi Dock"
+ },
+ {
+ "name": "LFT 1",
+ "pos_x": 0.65625,
+ "pos_y": -42.34375,
+ "pos_z": 9.46875,
+ "stations": "Rondon Prospect,Margulies Freeport"
+ },
+ {
+ "name": "LFT 1072",
+ "pos_x": -45.90625,
+ "pos_y": 43.71875,
+ "pos_z": -23.25,
+ "stations": "Fossum Depot,Matteucci City,Redi Plant"
+ },
+ {
+ "name": "LFT 1073",
+ "pos_x": -38.9375,
+ "pos_y": 32.5625,
+ "pos_z": -21.65625,
+ "stations": "Galois Installation,Cook Landing,Winne Installation"
+ },
+ {
+ "name": "LFT 1103",
+ "pos_x": 0.65625,
+ "pos_y": 40.625,
+ "pos_z": 22.71875,
+ "stations": "Kirk Ring,Nylund Reach,Sawyer Port"
+ },
+ {
+ "name": "LFT 1209",
+ "pos_x": -16.6875,
+ "pos_y": 51.5625,
+ "pos_z": 40,
+ "stations": "Patsayev Dock,Lovelace Terminal,Foale Orbital,Cabrera Installation"
+ },
+ {
+ "name": "LFT 1231",
+ "pos_x": -35.0625,
+ "pos_y": 51.78125,
+ "pos_z": 23.96875,
+ "stations": "Chilton Port,Anthony de la Roche City,Thompson Depot,Laird Vision,Plucker Depot"
+ },
+ {
+ "name": "LFT 1249",
+ "pos_x": -36.28125,
+ "pos_y": 48.53125,
+ "pos_z": 24.8125,
+ "stations": "Allen Terminal,Parazynski Survey"
+ },
+ {
+ "name": "LFT 1300",
+ "pos_x": 32.65625,
+ "pos_y": -16.03125,
+ "pos_z": 36.84375,
+ "stations": "Gulyaev Depot,Virchow Dock"
+ },
+ {
+ "name": "LFT 133",
+ "pos_x": 3.5625,
+ "pos_y": -59.875,
+ "pos_z": -7.25,
+ "stations": "Sullivan Dock,Garn Terminal,Horch Port,Burton Horizons"
+ },
+ {
+ "name": "LFT 1349",
+ "pos_x": 20.46875,
+ "pos_y": -10.8125,
+ "pos_z": 34.8125,
+ "stations": "Alas Refinery,Murray Platform,Johnson Keep"
+ },
+ {
+ "name": "LFT 140",
+ "pos_x": -27.875,
+ "pos_y": -3.1875,
+ "pos_z": -22.78125,
+ "stations": "Kafka Enterprise,Ford Orbital"
+ },
+ {
+ "name": "LFT 1404",
+ "pos_x": -25.84375,
+ "pos_y": 7.65625,
+ "pos_z": 44.1875,
+ "stations": "Russell Mines"
+ },
+ {
+ "name": "LFT 141",
+ "pos_x": 28.6875,
+ "pos_y": -67.1875,
+ "pos_z": 6.65625,
+ "stations": "Marius Horizons,Wilhelm Bastion,Hussenot Refinery"
+ },
+ {
+ "name": "LFT 142",
+ "pos_x": -8,
+ "pos_y": -36.40625,
+ "pos_z": -14.78125,
+ "stations": "DeLucas Camp,Rozhdestvensky Dock,Linteris Silo,Atkov Hub"
+ },
+ {
+ "name": "LFT 1421",
+ "pos_x": -45.46875,
+ "pos_y": 18.5625,
+ "pos_z": 12.59375,
+ "stations": "Ehrlich Orbital,Clark Enterprise,Wrangell Terminal,Illy Barracks"
+ },
+ {
+ "name": "LFT 1425",
+ "pos_x": -64.65625,
+ "pos_y": 20.5,
+ "pos_z": 36,
+ "stations": "Hirase Market"
+ },
+ {
+ "name": "LFT 1442",
+ "pos_x": 8.375,
+ "pos_y": -17,
+ "pos_z": 41.875,
+ "stations": "Harrison Port,West Gateway,Baturin Landing,Vaez de Torres Orbital,Brand Colony"
+ },
+ {
+ "name": "LFT 1446",
+ "pos_x": -38.8125,
+ "pos_y": 18.25,
+ "pos_z": -7.9375,
+ "stations": "Thorne Colony,Hurston Hub,White Arsenal"
+ },
+ {
+ "name": "LFT 1448",
+ "pos_x": -53.90625,
+ "pos_y": -0.6875,
+ "pos_z": 60.1875,
+ "stations": "Dirac Enterprise"
+ },
+ {
+ "name": "LFT 1492",
+ "pos_x": -41,
+ "pos_y": 2.53125,
+ "pos_z": 16.84375,
+ "stations": "Rushd Gateway"
+ },
+ {
+ "name": "LFT 1504",
+ "pos_x": -27.84375,
+ "pos_y": -21.625,
+ "pos_z": 49.6875,
+ "stations": "Brunel Terminal,Eyharts Hangar"
+ },
+ {
+ "name": "LFT 1594",
+ "pos_x": 10.78125,
+ "pos_y": -27.125,
+ "pos_z": 29.46875,
+ "stations": "Sinclair City"
+ },
+ {
+ "name": "LFT 1610",
+ "pos_x": 1.4375,
+ "pos_y": -33.46875,
+ "pos_z": 34.78125,
+ "stations": "Cormack Holdings,Hieb Horizons,Martin Enterprise"
+ },
+ {
+ "name": "LFT 163",
+ "pos_x": -7.28125,
+ "pos_y": -52.84375,
+ "pos_z": -20.78125,
+ "stations": "Dobrovolski Plant,Rukavishnikov Depot,Malenchenko Dock,Haller Plant,Erdos Landing"
+ },
+ {
+ "name": "LFT 1664",
+ "pos_x": -70.5,
+ "pos_y": -3.25,
+ "pos_z": -6.875,
+ "stations": "Shull Settlement,Teng-hui Colony"
+ },
+ {
+ "name": "LFT 1667",
+ "pos_x": -74.59375,
+ "pos_y": -13,
+ "pos_z": -1.875,
+ "stations": "Shkaplerov Mines"
+ },
+ {
+ "name": "LFT 1672",
+ "pos_x": 12.28125,
+ "pos_y": -28.46875,
+ "pos_z": 23.78125,
+ "stations": "McArthur Dock,Jensen Horizons"
+ },
+ {
+ "name": "LFT 168",
+ "pos_x": 2.84375,
+ "pos_y": -34.65625,
+ "pos_z": -9.03125,
+ "stations": "Barr Arsenal,Neff Station,Meucci Enterprise"
+ },
+ {
+ "name": "LFT 1723",
+ "pos_x": -30.59375,
+ "pos_y": -27,
+ "pos_z": 7.40625,
+ "stations": "Eyharts Orbital,Dutton City,Janes Keep,Linge Base,Morey City"
+ },
+ {
+ "name": "LFT 1739",
+ "pos_x": -63.1875,
+ "pos_y": -28.3125,
+ "pos_z": -4.875,
+ "stations": "Glazkov Hangar,Sacco Refinery"
+ },
+ {
+ "name": "LFT 174",
+ "pos_x": 3.6875,
+ "pos_y": -40.4375,
+ "pos_z": -12.125,
+ "stations": "Berezovoy Gateway,Divis Park,Wescott Orbital"
+ },
+ {
+ "name": "LFT 1748",
+ "pos_x": -43.375,
+ "pos_y": 1,
+ "pos_z": -15.125,
+ "stations": "Otiman Dock,Chomsky Enterprise,Westerfeld Enterprise,Adams City,Ehrlich Orbital,Carpenter City,Tito Enterprise,Kanwar City"
+ },
+ {
+ "name": "LFT 1749",
+ "pos_x": -58.875,
+ "pos_y": -100.3125,
+ "pos_z": 28.875,
+ "stations": "Keyes Port,Brosnan Beacon"
+ },
+ {
+ "name": "LFT 1834",
+ "pos_x": 9.78125,
+ "pos_y": -79.28125,
+ "pos_z": 24.8125,
+ "stations": "Margulies Port,Andrews Enterprise,Cadamosto Prospect,Shalatula Orbital,Galiano's Progress,Mohri Vision,Bellamy Dock,Witt Works"
+ },
+ {
+ "name": "LFT 214",
+ "pos_x": -37.65625,
+ "pos_y": -2.78125,
+ "pos_z": -39.125,
+ "stations": "Molina Relay,Payette Vision,Fozard Penal colony"
+ },
+ {
+ "name": "LFT 269",
+ "pos_x": -52.3125,
+ "pos_y": 3.875,
+ "pos_z": -62.4375,
+ "stations": "Morukov Colony"
+ },
+ {
+ "name": "LFT 300",
+ "pos_x": -16.9375,
+ "pos_y": -27.21875,
+ "pos_z": -59.9375,
+ "stations": "Wedge Hangar,Jones Depot,Blodgett Plant,Hahn Vision,Petaja Vision"
+ },
+ {
+ "name": "LFT 361",
+ "pos_x": -45.875,
+ "pos_y": 9.90625,
+ "pos_z": -84.53125,
+ "stations": "Curie Terminal,Vinge Camp,Valdes Installation,Clifford Enterprise,Hope Gateway,Macarthur Dock,Franklin Landing"
+ },
+ {
+ "name": "LFT 367",
+ "pos_x": 11,
+ "pos_y": -17.21875,
+ "pos_z": -23.40625,
+ "stations": "Levi-Montalcini Terminal,Acton Dock,Adams Station,Kagan Enterprise,Cook's Progress,Roentgen Silo"
+ },
+ {
+ "name": "LFT 37",
+ "pos_x": 14.4375,
+ "pos_y": -61.875,
+ "pos_z": 16.5,
+ "stations": "Roentgen Hub,Savinykh Hub,Onnes Gateway,Cabana Barracks"
+ },
+ {
+ "name": "LFT 374",
+ "pos_x": 50.625,
+ "pos_y": -43.90625,
+ "pos_z": -11.84375,
+ "stations": "Volterra Terminal,Schilling Vision,Brooks Vision,Kooi Refinery"
+ },
+ {
+ "name": "LFT 392",
+ "pos_x": 7.75,
+ "pos_y": -16.90625,
+ "pos_z": -65.375,
+ "stations": "Tudela Installation"
+ },
+ {
+ "name": "LFT 415",
+ "pos_x": 25.8125,
+ "pos_y": -20.71875,
+ "pos_z": -51.78125,
+ "stations": "Lazarev Port,Descartes City"
+ },
+ {
+ "name": "LFT 42",
+ "pos_x": -42.96875,
+ "pos_y": -39.21875,
+ "pos_z": -20.15625,
+ "stations": "Bennett Vision,Spring Plant,Phillips Survey,Grant Relay,Crown Landing"
+ },
+ {
+ "name": "LFT 424",
+ "pos_x": -14.46875,
+ "pos_y": 8.8125,
+ "pos_z": -37.03125,
+ "stations": "Coke Dock,Howe Park,Lowry Observatory,Diesel Orbital"
+ },
+ {
+ "name": "LFT 434",
+ "pos_x": 38.3125,
+ "pos_y": -22.65625,
+ "pos_z": -20.53125,
+ "stations": "Vinogradov Enterprise,Cooper Terminal,Daimler Gateway,Haberlandt's Progress"
+ },
+ {
+ "name": "LFT 444",
+ "pos_x": 41.1875,
+ "pos_y": -21.28125,
+ "pos_z": -26.65625,
+ "stations": "Serebrov Station"
+ },
+ {
+ "name": "LFT 446",
+ "pos_x": -18.125,
+ "pos_y": 15.46875,
+ "pos_z": -68.46875,
+ "stations": "Francisco de Eliza Ring,Turtledove Horizons,Mach Installation,Young Silo,Woolley Orbital"
+ },
+ {
+ "name": "LFT 551",
+ "pos_x": 54.5625,
+ "pos_y": 6.8125,
+ "pos_z": -43.0625,
+ "stations": "Agassiz Hangar"
+ },
+ {
+ "name": "LFT 563",
+ "pos_x": 59.34375,
+ "pos_y": -5.71875,
+ "pos_z": -15.21875,
+ "stations": "Ford Refinery,Tyson Dock,Cousteau Barracks"
+ },
+ {
+ "name": "LFT 568",
+ "pos_x": 53.1875,
+ "pos_y": -9.90625,
+ "pos_z": -1.9375,
+ "stations": "Ramaswamy Outpost"
+ },
+ {
+ "name": "LFT 6",
+ "pos_x": -32.65625,
+ "pos_y": -10.4375,
+ "pos_z": -15.28125,
+ "stations": "Lamarck Colony,Mayer Plant,Larson Base"
+ },
+ {
+ "name": "LFT 601",
+ "pos_x": 26.65625,
+ "pos_y": 24.8125,
+ "pos_z": -34.75,
+ "stations": "Hilmers Market,Scheutz Hub,Cunningham Terminal,Carey Terminal,Bykovsky Horizons,Feustel Prospect,Nyberg Hub,Barton Beacon,McArthur Terminal"
+ },
+ {
+ "name": "LFT 619",
+ "pos_x": 29.96875,
+ "pos_y": 49.96875,
+ "pos_z": -59.71875,
+ "stations": "White Base,Payette Colony,Parsons Horizons"
+ },
+ {
+ "name": "LFT 625",
+ "pos_x": 50.21875,
+ "pos_y": 8.71875,
+ "pos_z": -13,
+ "stations": "Fox Enterprise,Newman Silo"
+ },
+ {
+ "name": "LFT 682",
+ "pos_x": 32,
+ "pos_y": 3.1875,
+ "pos_z": 1.46875,
+ "stations": "Gernhardt Vision,Crouch Reach"
+ },
+ {
+ "name": "LFT 69",
+ "pos_x": 6.15625,
+ "pos_y": -31.3125,
+ "pos_z": 5,
+ "stations": "Anning Enterprise,Parise Laboratory,Franklin Prospect"
+ },
+ {
+ "name": "LFT 698",
+ "pos_x": 27.25,
+ "pos_y": 26.75,
+ "pos_z": -12.625,
+ "stations": "Ivanishin Settlement,Ampere Colony,Curie Depot"
+ },
+ {
+ "name": "LFT 709",
+ "pos_x": 34.6875,
+ "pos_y": 26.15625,
+ "pos_z": -9.46875,
+ "stations": "Hutchinson Ring,Marley Port,Hui Hub,Kiernan Station,Cobb Enterprise,Sohl Hub,Malchiodi City,Bellamy Gateway,Hand Terminal,Carey Terminal,Mieville Base"
+ },
+ {
+ "name": "LFT 78",
+ "pos_x": 33,
+ "pos_y": -58.875,
+ "pos_z": 21.71875,
+ "stations": "Coye Orbital,Hale Orbital,Hilbert Lab,Niijima Silo,Kirkwood Terminal"
+ },
+ {
+ "name": "LFT 820",
+ "pos_x": 12.34375,
+ "pos_y": 54.5,
+ "pos_z": -13.5625,
+ "stations": "Michelson Hub,Holub Hub,Olivas Hub,Adams Beacon,Pippin Settlement"
+ },
+ {
+ "name": "LFT 824",
+ "pos_x": 45.96875,
+ "pos_y": 33.71875,
+ "pos_z": 9.71875,
+ "stations": "Effinger Reach"
+ },
+ {
+ "name": "LFT 859",
+ "pos_x": 12.5625,
+ "pos_y": 31.46875,
+ "pos_z": -1.71875,
+ "stations": "Guidoni Orbital,Scobee Enterprise,Bouch Hangar"
+ },
+ {
+ "name": "LFT 880",
+ "pos_x": -22.8125,
+ "pos_y": 31.40625,
+ "pos_z": -18.34375,
+ "stations": "Baker Settlement,Baker Platform"
+ },
+ {
+ "name": "LFT 90",
+ "pos_x": -29.21875,
+ "pos_y": -0.9375,
+ "pos_z": -19.8125,
+ "stations": "Teller Ring,Gregory Ring,Yamazaki Penal colony,Bohm Beacon"
+ },
+ {
+ "name": "LFT 926",
+ "pos_x": 51.03125,
+ "pos_y": 17.6875,
+ "pos_z": 30.21875,
+ "stations": "Meredith City,Dirichlet Depot"
+ },
+ {
+ "name": "LFT 945",
+ "pos_x": 27.5,
+ "pos_y": 53.90625,
+ "pos_z": 16.40625,
+ "stations": "Robinson Vision,Anthony's Inheritance"
+ },
+ {
+ "name": "LFT 992",
+ "pos_x": -7.5625,
+ "pos_y": 42.59375,
+ "pos_z": 0.6875,
+ "stations": "Szulkin Mines,Wilson Vision"
+ },
+ {
+ "name": "Lhan",
+ "pos_x": 56.15625,
+ "pos_y": -16.34375,
+ "pos_z": -42.65625,
+ "stations": "Johnson Vision"
+ },
+ {
+ "name": "Lhana",
+ "pos_x": 70.75,
+ "pos_y": 29.40625,
+ "pos_z": -48.75,
+ "stations": "Bresnik Station,Flade Enterprise,Sagan Orbital,Thompson Hub,Edwards Hub,Galvani Dock,Irvin Port,Reynolds Terminal,Kwolek Terminal,Trinh Depot,Geston Terminal,Dutton Gateway,Houtman Point"
+ },
+ {
+ "name": "Lhanayi",
+ "pos_x": 174.375,
+ "pos_y": 3.25,
+ "pos_z": 67.6875,
+ "stations": "Hopkinson Gateway,Plucker Depot,Steakley Vision"
+ },
+ {
+ "name": "Lhangkambe",
+ "pos_x": -67.125,
+ "pos_y": 20.9375,
+ "pos_z": 14.875,
+ "stations": "Yeliseyev Terminal,Jacquard Dock,Detmer Orbital,Baker Orbital,Glidden Gateway"
+ },
+ {
+ "name": "Lhanla",
+ "pos_x": -57.40625,
+ "pos_y": -105,
+ "pos_z": 115.96875,
+ "stations": "Thompson Vision,Kettle Colony"
+ },
+ {
+ "name": "Lhou Ling",
+ "pos_x": 115.4375,
+ "pos_y": -96.6875,
+ "pos_z": -54.6875,
+ "stations": "Gonnessiat Port,Fabian Terminal,McQuarrie Station,Alexandria Installation,Engle Forum"
+ },
+ {
+ "name": "Lhou Mans",
+ "pos_x": -97.21875,
+ "pos_y": 34.875,
+ "pos_z": -83.125,
+ "stations": "Webb City,Ryman Enterprise,Sabine Gateway,Dashiell Port,Ellison City,Baudin Gateway,Laumer Station,Napier Bastion,Springer Relay,Rotsler Ring,Brust Forum,Russell Gateway,Kennan Port"
+ },
+ {
+ "name": "Lhou Tiegua",
+ "pos_x": -123.53125,
+ "pos_y": -28.3125,
+ "pos_z": -88.4375,
+ "stations": "Leclerc Works"
+ },
+ {
+ "name": "Lhou Wangs",
+ "pos_x": -37.1875,
+ "pos_y": -1.0625,
+ "pos_z": -123.15625,
+ "stations": "Ride Base,Bolotov Landing"
+ },
+ {
+ "name": "Lhou Xuang",
+ "pos_x": 131.5625,
+ "pos_y": -82.21875,
+ "pos_z": 19.125,
+ "stations": "Giraud Observatory,Riess Outpost"
+ },
+ {
+ "name": "LHS 1004",
+ "pos_x": -61.4375,
+ "pos_y": -46.46875,
+ "pos_z": -21.4375,
+ "stations": "Garrett Mines,Whitney Base"
+ },
+ {
+ "name": "LHS 1020",
+ "pos_x": 20.78125,
+ "pos_y": -77.125,
+ "pos_z": 25.625,
+ "stations": "Corbusier Market,Arfstrom City,Babakin Terminal,Ziewe Terminal"
+ },
+ {
+ "name": "LHS 103",
+ "pos_x": -47.78125,
+ "pos_y": -32.90625,
+ "pos_z": -18.84375,
+ "stations": "Oliver Beacon,Snodgrass Terminal,Bryant Orbital,Alexander Terminal"
+ },
+ {
+ "name": "LHS 1050",
+ "pos_x": -23.625,
+ "pos_y": -28.28125,
+ "pos_z": -8.53125,
+ "stations": "Apgar Horizons,So-yeon Terminal,Hale Port"
+ },
+ {
+ "name": "LHS 1052",
+ "pos_x": -30.25,
+ "pos_y": -48.15625,
+ "pos_z": -9.34375,
+ "stations": "Vinge's Claim"
+ },
+ {
+ "name": "LHS 1065",
+ "pos_x": -51.71875,
+ "pos_y": 15.84375,
+ "pos_z": -31.75,
+ "stations": "Nelson Station,Freycinet Port,Hedin Silo,Flynn Beacon,Pratchett Gateway"
+ },
+ {
+ "name": "LHS 1067",
+ "pos_x": -3.3125,
+ "pos_y": -59.1875,
+ "pos_z": 4.59375,
+ "stations": "Kuhn Port,Dutton Station,Yurchikhin Station,Shapiro Barracks,Gamow Station,Onufriyenko Market,Crampton Terminal,Brackett Camp,Currie Terminal,Freud Port,Selye Port,Guidoni Terminal"
+ },
+ {
+ "name": "LHS 1071",
+ "pos_x": 34.3125,
+ "pos_y": -103.90625,
+ "pos_z": 31.28125,
+ "stations": "Gibson La Lisa Station,Mitanek Orbiter,Parkinson's Claim,Trumpler Colony,Verrier Horizons"
+ },
+ {
+ "name": "LHS 1097",
+ "pos_x": 8.84375,
+ "pos_y": -106.90625,
+ "pos_z": 12.75,
+ "stations": "Arnason Gateway,Wilson Depot,Amnuel Dock,Tanner Enterprise"
+ },
+ {
+ "name": "LHS 1101",
+ "pos_x": -54.46875,
+ "pos_y": 9.375,
+ "pos_z": -33.46875,
+ "stations": "Clement Hub,Zamka Station,Helms Ring,Ray Gateway"
+ },
+ {
+ "name": "LHS 1106",
+ "pos_x": -31.6875,
+ "pos_y": -104.71875,
+ "pos_z": -13.25,
+ "stations": "Salam Point"
+ },
+ {
+ "name": "LHS 1110",
+ "pos_x": 16.4375,
+ "pos_y": -132.21875,
+ "pos_z": 17.125,
+ "stations": "Brill Ring,Iben City,Cottenot City,Gaensler Ring,Tem Plant,South Survey,MacCready Terminal,Samuda Depot,de Seversky Hub,Fehrenbach Hub"
+ },
+ {
+ "name": "LHS 112",
+ "pos_x": -32.15625,
+ "pos_y": -20,
+ "pos_z": -15.34375,
+ "stations": "Hale Port,Weitz Orbital,Birdseye Terminal,Oefelein Station,Edgeworth Port,Parise Port,Surayev Ring,Polansky Orbital,Strzelecki Vision,Cormack Horizons,Lethem Base,Brongniart Ring"
+ },
+ {
+ "name": "LHS 1122",
+ "pos_x": 17.8125,
+ "pos_y": -74.84375,
+ "pos_z": 14.1875,
+ "stations": "Maupertuis City,Halley Orbital,Laurent Orbital,Nelson's Inheritance,Griffith Gateway"
+ },
+ {
+ "name": "LHS 115",
+ "pos_x": -27.9375,
+ "pos_y": 2.65625,
+ "pos_z": -17.0625,
+ "stations": "Aleksandrov Gateway"
+ },
+ {
+ "name": "LHS 1163",
+ "pos_x": 2.375,
+ "pos_y": -46.1875,
+ "pos_z": 0.6875,
+ "stations": "Hunziker Terminal,Carson Enterprise,Rennie Orbital,Dias Bastion,Gerst Works"
+ },
+ {
+ "name": "LHS 1167",
+ "pos_x": -65.90625,
+ "pos_y": 8.9375,
+ "pos_z": -44,
+ "stations": "Ahern Lab,Fangrim's Folly,Chilton Hub"
+ },
+ {
+ "name": "LHS 1197",
+ "pos_x": 2.5625,
+ "pos_y": -42.8125,
+ "pos_z": -1.625,
+ "stations": "McArthur Dock,Chretien Terminal,Piaget Orbital,White Relay"
+ },
+ {
+ "name": "LHS 120",
+ "pos_x": -44.53125,
+ "pos_y": -35.5,
+ "pos_z": -26.59375,
+ "stations": "Rolland Station,Vespucci Station"
+ },
+ {
+ "name": "LHS 1212",
+ "pos_x": -20,
+ "pos_y": -42.625,
+ "pos_z": -18.3125,
+ "stations": "Bramah Port,Menezes Landing,Foale Terminal,Ashby Landing"
+ },
+ {
+ "name": "LHS 1226",
+ "pos_x": -25.65625,
+ "pos_y": -55.65625,
+ "pos_z": -26.65625,
+ "stations": "Carter Horizons,Bardeen Vision,Anderson Beacon"
+ },
+ {
+ "name": "LHS 1228",
+ "pos_x": -10.0625,
+ "pos_y": -69.375,
+ "pos_z": -17.21875,
+ "stations": "Cooper City,Lavoisier Hub,Walker Terminal,Sacco Bastion,Culpeper Relay"
+ },
+ {
+ "name": "LHS 1230",
+ "pos_x": -26.03125,
+ "pos_y": -76.6875,
+ "pos_z": -30.21875,
+ "stations": "Ohm Port,Drebbel City,Popov Keep,Bardeen Base,Altshuller Relay"
+ },
+ {
+ "name": "LHS 1240",
+ "pos_x": -14.71875,
+ "pos_y": -41.375,
+ "pos_z": -17.1875,
+ "stations": "Hewish Installation,Ferguson Hub,Koch Enterprise,Szilard Gateway"
+ },
+ {
+ "name": "LHS 1246",
+ "pos_x": -53.71875,
+ "pos_y": -71.21875,
+ "pos_z": -53.21875,
+ "stations": "Parker Terminal,Spring Platform,Karl Diesel Terminal"
+ },
+ {
+ "name": "LHS 1275",
+ "pos_x": -61,
+ "pos_y": -57.78125,
+ "pos_z": -63.9375,
+ "stations": "Nagel Legacy,Gooch Mine"
+ },
+ {
+ "name": "LHS 1339",
+ "pos_x": 6.46875,
+ "pos_y": -28.9375,
+ "pos_z": -6.0625,
+ "stations": "Bosch City,Laue Terminal,Schlegel Port"
+ },
+ {
+ "name": "LHS 134",
+ "pos_x": -3.28125,
+ "pos_y": -32.1875,
+ "pos_z": -4.28125,
+ "stations": "Alas City,Helmholtz's Folly,Treshchov Hub,Skvortsov Terminal"
+ },
+ {
+ "name": "LHS 1348",
+ "pos_x": -41.96875,
+ "pos_y": -30.40625,
+ "pos_z": -50.9375,
+ "stations": "Haxel Terminal,Ramon Dock,Lebedev Hub,Detmer Orbital,Jacobi Enterprise,Bresnik Dock,Galilei Port"
+ },
+ {
+ "name": "LHS 135",
+ "pos_x": -27.1875,
+ "pos_y": -23.09375,
+ "pos_z": -20.25,
+ "stations": "Pangborn City,Carver Dock,Bombelli Hub,Pelt Prospect,Culpeper Exchange,Fadlan Port,Kagan Orbital,Gregory Dock,Macgregor Hub,Burstein Terminal,Potagos Orbital,Asaro Terminal"
+ },
+ {
+ "name": "LHS 1358",
+ "pos_x": -9.25,
+ "pos_y": -45.375,
+ "pos_z": -28.53125,
+ "stations": "Seega Landing,Davy Beacon"
+ },
+ {
+ "name": "LHS 137",
+ "pos_x": -51.59375,
+ "pos_y": -35.03125,
+ "pos_z": -38.5625,
+ "stations": "Casper Colony,Agnesi Hub,Nelson Silo"
+ },
+ {
+ "name": "LHS 1379",
+ "pos_x": 38.34375,
+ "pos_y": -64.03125,
+ "pos_z": 5.125,
+ "stations": "Prunariu Landing,Grabe Plant,O'Brien Base"
+ },
+ {
+ "name": "LHS 1380",
+ "pos_x": 29,
+ "pos_y": -87.40625,
+ "pos_z": -15.40625,
+ "stations": "Zel'dovich Vision,Sarmiento de Gamboa Base,Gurevich Orbital,Tolstoi's Progress"
+ },
+ {
+ "name": "LHS 1387",
+ "pos_x": 7.9375,
+ "pos_y": -38.8125,
+ "pos_z": -11.8125,
+ "stations": "Angel's Sunshine,Ataturk Station,Slonczewski Platform,Galois' Inheritance"
+ },
+ {
+ "name": "LHS 139",
+ "pos_x": -40.40625,
+ "pos_y": -41.59375,
+ "pos_z": -34.125,
+ "stations": "Watt Orbital,Ray Station,Andrews Observatory,Fossum Base"
+ },
+ {
+ "name": "LHS 1391",
+ "pos_x": 27.9375,
+ "pos_y": -100.15625,
+ "pos_z": -24.75,
+ "stations": "Bowen Relay,McQuarrie Station,Kafka Base,Lagrange Refinery"
+ },
+ {
+ "name": "LHS 1393",
+ "pos_x": -39.875,
+ "pos_y": -27.09375,
+ "pos_z": -51.40625,
+ "stations": "Wyeth Dock,Baird City,Cabana Keep,Starzl Installation,Brady Station"
+ },
+ {
+ "name": "LHS 140",
+ "pos_x": -34.53125,
+ "pos_y": 16.1875,
+ "pos_z": -23.0625,
+ "stations": "Lawhead Installation,Mondeh Station,Pippin City"
+ },
+ {
+ "name": "LHS 142",
+ "pos_x": 1.21875,
+ "pos_y": -57.15625,
+ "pos_z": -11.34375,
+ "stations": "Kregel Enterprise,Kozeyev Orbital,Watson Station,Noriega Port,Weil's Claim,Haignere Station,vo Dock,Grandin Dock,Weitz Port,Bendell Prospect,Fearn Point,MacVicar Vision,Belyayev Port,Forest Landing"
+ },
+ {
+ "name": "LHS 1442",
+ "pos_x": -2.875,
+ "pos_y": -54.65625,
+ "pos_z": -41.03125,
+ "stations": "Ham Vision,Lawson Platform,Polyakov Depot,Vanguard"
+ },
+ {
+ "name": "LHS 1446",
+ "pos_x": -21.75,
+ "pos_y": -2.75,
+ "pos_z": -25.53125,
+ "stations": "Blalock Orbital,Ackerman Base,Goddard Survey"
+ },
+ {
+ "name": "LHS 145",
+ "pos_x": 24.40625,
+ "pos_y": -31.21875,
+ "pos_z": 11.5625,
+ "stations": "Babbage Keep,Finney Settlement,de Balboa Hub"
+ },
+ {
+ "name": "LHS 1453",
+ "pos_x": -19.0625,
+ "pos_y": -45.4375,
+ "pos_z": -55.4375,
+ "stations": "Saavedra Port,Jakes Ring,Romanenko Enterprise,Bisson Refinery"
+ },
+ {
+ "name": "LHS 1483",
+ "pos_x": -29.28125,
+ "pos_y": -20.59375,
+ "pos_z": -50.09375,
+ "stations": "Bykovsky Ring,Litke Arsenal,Nicollier Ring,Vaucanson Settlement"
+ },
+ {
+ "name": "LHS 1505",
+ "pos_x": -50.25,
+ "pos_y": 18.8125,
+ "pos_z": -44.09375,
+ "stations": "Arkwright Horizons"
+ },
+ {
+ "name": "LHS 1507",
+ "pos_x": -27.5,
+ "pos_y": -9.125,
+ "pos_z": -42.5,
+ "stations": "Gibson Settlement,Gubarev Hub,Euler Port"
+ },
+ {
+ "name": "LHS 1541",
+ "pos_x": -23.78125,
+ "pos_y": -10.8125,
+ "pos_z": -42.53125,
+ "stations": "Strekalov Dock,Haller Port,Foda Station,Hennen City,Archimedes Hub,Pennington City,Cabrera Dock,Stebler City,Ross Dock,Boodt Plant,Linge Holdings,Fontana Point"
+ },
+ {
+ "name": "LHS 1547",
+ "pos_x": 3.21875,
+ "pos_y": -37.4375,
+ "pos_z": -36.84375,
+ "stations": "Sherrington Station,Otiman Station,Khan Dock,Foucault Hub,Wallace Hub,Kelly Port,Godwin Orbital,Bernard Hub,Worlidge Station,Spielberg Installation,H. G. Wells Beacon"
+ },
+ {
+ "name": "LHS 154a",
+ "pos_x": 15.03125,
+ "pos_y": -42.8125,
+ "pos_z": -7.0625,
+ "stations": "Evans City,Edwards Depot,Fung Station,Archambault Port,Pournelle's Inheritance"
+ },
+ {
+ "name": "LHS 1561",
+ "pos_x": 6.3125,
+ "pos_y": -35.09375,
+ "pos_z": -34.25,
+ "stations": "Belyayev Relay,Nicollier Terminal,Deb Orbital"
+ },
+ {
+ "name": "LHS 1567",
+ "pos_x": 42.125,
+ "pos_y": -62.5,
+ "pos_z": -18.6875,
+ "stations": "Kuo Colony,Pirsan Works"
+ },
+ {
+ "name": "LHS 1573",
+ "pos_x": -15.09375,
+ "pos_y": -24.21875,
+ "pos_z": -53.28125,
+ "stations": "DG-1 Refinery,Blackman Terminal"
+ },
+ {
+ "name": "LHS 1590",
+ "pos_x": 19.4375,
+ "pos_y": -57.21875,
+ "pos_z": -51.78125,
+ "stations": "Okorafor Hangar,Leichhardt Plant,Thagard's Inheritance,Archer Enterprise"
+ },
+ {
+ "name": "LHS 1599",
+ "pos_x": 10.8125,
+ "pos_y": -69.1875,
+ "pos_z": -88,
+ "stations": "Zamyatin Settlement,Mohmand Silo,Chebyshev Platform"
+ },
+ {
+ "name": "LHS 160",
+ "pos_x": 24.46875,
+ "pos_y": -28.53125,
+ "pos_z": 5.59375,
+ "stations": "Jun Orbital,Crown Orbital,Tarski Depot,Crown City"
+ },
+ {
+ "name": "LHS 1604",
+ "pos_x": 5.90625,
+ "pos_y": -30.5,
+ "pos_z": -36.375,
+ "stations": "Gernsback City,Rocklynne Orbital,Montrose Gateway,Nourse Relay,May Base"
+ },
+ {
+ "name": "LHS 1612",
+ "pos_x": -40.4375,
+ "pos_y": 7.40625,
+ "pos_z": -54.875,
+ "stations": "Wafer Terminal,Frobenius Station,Bulgakov Orbital,Bryusov Survey"
+ },
+ {
+ "name": "LHS 1617",
+ "pos_x": 1.78125,
+ "pos_y": -30.65625,
+ "pos_z": -48.6875,
+ "stations": "Lebedev Hub,Hennen Gateway,Faraday Station,Comino Hub,Malpighi Port,Ejeta Terminal,Conti Settlement,Leslie Survey,Whitney Relay,Yang Orbital,Behring Station,Stackpole Installation"
+ },
+ {
+ "name": "LHS 1650",
+ "pos_x": 30.1875,
+ "pos_y": -29.6875,
+ "pos_z": -4.21875,
+ "stations": "Jonah Musry,Campbell Vision,SDM Galactic Starpost,Gagarin Landing"
+ },
+ {
+ "name": "LHS 1651",
+ "pos_x": 31.25,
+ "pos_y": -44.09375,
+ "pos_z": -32.5625,
+ "stations": "Camarda Vision,Teng-hui Hangar,Eckford Survey"
+ },
+ {
+ "name": "LHS 1663",
+ "pos_x": -40.53125,
+ "pos_y": 18.25,
+ "pos_z": -40.90625,
+ "stations": "Bulychev Holdings,Frobisher City,O'Donnell Gateway"
+ },
+ {
+ "name": "LHS 1681",
+ "pos_x": -2.46875,
+ "pos_y": -15.78125,
+ "pos_z": -48.40625,
+ "stations": "Anning Port"
+ },
+ {
+ "name": "LHS 17",
+ "pos_x": -0.5625,
+ "pos_y": -43.71875,
+ "pos_z": -30.8125,
+ "stations": "Song Point,Morgan Landing,Fuca Beacon"
+ },
+ {
+ "name": "LHS 1706",
+ "pos_x": -1.125,
+ "pos_y": -11.09375,
+ "pos_z": -44.3125,
+ "stations": "Reiter Terminal"
+ },
+ {
+ "name": "LHS 1743",
+ "pos_x": 5.59375,
+ "pos_y": -16.125,
+ "pos_z": -73.9375,
+ "stations": "Tyurin Ring,Jemison Ring,Potter Gateway"
+ },
+ {
+ "name": "LHS 1748",
+ "pos_x": 46.1875,
+ "pos_y": -37.0625,
+ "pos_z": -33.3125,
+ "stations": "Bernoulli Dock,Woolley Installation"
+ },
+ {
+ "name": "LHS 1758",
+ "pos_x": -38.125,
+ "pos_y": 17.71875,
+ "pos_z": -72.3125,
+ "stations": "Gaiman's Inheritance"
+ },
+ {
+ "name": "LHS 1768",
+ "pos_x": 51.25,
+ "pos_y": -36.3125,
+ "pos_z": -80.09375,
+ "stations": "Manakov Horizons,Hopkins Hangar,Ashby Depot"
+ },
+ {
+ "name": "LHS 1779",
+ "pos_x": 40.53125,
+ "pos_y": -24.90625,
+ "pos_z": -96.53125,
+ "stations": "Murray Base,Clement Dock"
+ },
+ {
+ "name": "LHS 1788",
+ "pos_x": -42.8125,
+ "pos_y": 26.375,
+ "pos_z": -58.21875,
+ "stations": "Budrys Beacon,Khan Terminal,McArthur Colony"
+ },
+ {
+ "name": "LHS 1794",
+ "pos_x": 5.3125,
+ "pos_y": -1.03125,
+ "pos_z": -62.25,
+ "stations": "Bruce Installation,Ricardo Landing"
+ },
+ {
+ "name": "LHS 1798",
+ "pos_x": 35.53125,
+ "pos_y": -16.65625,
+ "pos_z": -77.28125,
+ "stations": "Balmer Base,Collins Vision,Korzun Landing"
+ },
+ {
+ "name": "LHS 1803",
+ "pos_x": 21.65625,
+ "pos_y": -7.53125,
+ "pos_z": -83.6875,
+ "stations": "Rontgen Colony,Agnesi Settlement,Tesla's Inheritance"
+ },
+ {
+ "name": "LHS 1809",
+ "pos_x": -8.53125,
+ "pos_y": 6.84375,
+ "pos_z": -28.21875,
+ "stations": "Nowak Landing"
+ },
+ {
+ "name": "LHS 1832",
+ "pos_x": 80.375,
+ "pos_y": -44.0625,
+ "pos_z": 6.84375,
+ "stations": "Coney Gateway,Holub Vision,Dalmas City,Cantor's Folly"
+ },
+ {
+ "name": "LHS 184",
+ "pos_x": 34.375,
+ "pos_y": -48.78125,
+ "pos_z": -20.5,
+ "stations": "Cremona Settlement,Tarski Port,Veblen Colony,Bruce Depot"
+ },
+ {
+ "name": "LHS 1848",
+ "pos_x": -24.96875,
+ "pos_y": 23.5,
+ "pos_z": -64.0625,
+ "stations": "Gagarin Dock,Bacigalupi Survey,Aubakirov Installation"
+ },
+ {
+ "name": "LHS 1852",
+ "pos_x": 68.0625,
+ "pos_y": -35.25,
+ "pos_z": 12.46875,
+ "stations": "Finlay-Freundlich Platform,Herbert's Folly,Hamuy City"
+ },
+ {
+ "name": "LHS 1857",
+ "pos_x": 21.03125,
+ "pos_y": 1.96875,
+ "pos_z": -55.3125,
+ "stations": "Planck Dock,Apt Camp,Dirac Hub"
+ },
+ {
+ "name": "LHS 1875",
+ "pos_x": 17.46875,
+ "pos_y": -0.84375,
+ "pos_z": -22.40625,
+ "stations": "Sylvester Dock,Malaspina Station,Grzimek Hub,Kozeyev Landing,Cunningham Silo"
+ },
+ {
+ "name": "LHS 1882",
+ "pos_x": -16.4375,
+ "pos_y": 21.375,
+ "pos_z": -48.6875,
+ "stations": "Brand Landing,Arber Dock"
+ },
+ {
+ "name": "LHS 1885",
+ "pos_x": -10.21875,
+ "pos_y": 10.5,
+ "pos_z": -20.625,
+ "stations": "Sherrington Orbital"
+ },
+ {
+ "name": "LHS 1887",
+ "pos_x": 43.5,
+ "pos_y": -3.3125,
+ "pos_z": -46.75,
+ "stations": "Abnett Refinery,Zebrowski Station,Clair Terminal,Barton Vision"
+ },
+ {
+ "name": "LHS 191",
+ "pos_x": 9.09375,
+ "pos_y": -27.625,
+ "pos_z": -47.375,
+ "stations": "Zamka Station,Brunton Ring,Hoffman Dock"
+ },
+ {
+ "name": "LHS 1914",
+ "pos_x": 9.84375,
+ "pos_y": 26.15625,
+ "pos_z": -72.46875,
+ "stations": "Payton Orbital,Galvani Port,Heisenberg Terminal,Arnarson Beacon,Bohm Terminal,Vittori Terminal,Bosch Terminal,Scheutz Hub,Volta Dock,Schirra Orbital,Jahn Vista,Adamson Enterprise,MacLean Settlement,Simak Horizons,Bagian Depot"
+ },
+ {
+ "name": "LHS 1918",
+ "pos_x": 30.5625,
+ "pos_y": -0.5,
+ "pos_z": -22.4375,
+ "stations": "Haller Prospect,Walker Station,Atiyah Legacy,Euclid Terminal,Bosch Terminal"
+ },
+ {
+ "name": "LHS 1920",
+ "pos_x": 25.84375,
+ "pos_y": 5.0625,
+ "pos_z": -30.21875,
+ "stations": "McArthur Plant,Tsibliyev Hangar"
+ },
+ {
+ "name": "LHS 1923",
+ "pos_x": -18.59375,
+ "pos_y": 27.84375,
+ "pos_z": -48.96875,
+ "stations": "Shull Colony,Tarelkin Terminal,Vancouver Vision"
+ },
+ {
+ "name": "LHS 1928",
+ "pos_x": 28.3125,
+ "pos_y": 8.28125,
+ "pos_z": -37.65625,
+ "stations": "Treshchov Installation,Polyakov Dock,Tombaugh Dock,Tombaugh Barracks"
+ },
+ {
+ "name": "LHS 1933",
+ "pos_x": 15,
+ "pos_y": 30.71875,
+ "pos_z": -74.71875,
+ "stations": "Shatalov Prospect,Hernandez Relay,Herodotus Vision"
+ },
+ {
+ "name": "LHS 1944",
+ "pos_x": 0.5625,
+ "pos_y": 51.71875,
+ "pos_z": -100.3125,
+ "stations": "Altman Terminal,Bakewell Gateway"
+ },
+ {
+ "name": "LHS 1951",
+ "pos_x": 17.875,
+ "pos_y": 6.625,
+ "pos_z": -21.375,
+ "stations": "Hobaugh Orbital,Gibson Orbital,Plexico Survey,Faris Relay,Sanger Point"
+ },
+ {
+ "name": "LHS 1963",
+ "pos_x": -0.4375,
+ "pos_y": 14.09375,
+ "pos_z": -24.3125,
+ "stations": "Hardy Hub,Arnarson Horizons,Bunch Hub"
+ },
+ {
+ "name": "LHS 197",
+ "pos_x": -24.34375,
+ "pos_y": 2.375,
+ "pos_z": -58.03125,
+ "stations": "Yu Port,Dedekind Arsenal,Meaney Horizons"
+ },
+ {
+ "name": "LHS 199",
+ "pos_x": 96,
+ "pos_y": -73.34375,
+ "pos_z": 1.375,
+ "stations": "Harawi Hub,Piano Station"
+ },
+ {
+ "name": "LHS 20",
+ "pos_x": 11.1875,
+ "pos_y": -37.375,
+ "pos_z": -31.84375,
+ "stations": "Ohm City,Jacobi Silo,Lazutkin Terminal"
+ },
+ {
+ "name": "LHS 200",
+ "pos_x": 10.84375,
+ "pos_y": -12.71875,
+ "pos_z": -22.96875,
+ "stations": "Tsunenaga Port,Herodotus Penal colony"
+ },
+ {
+ "name": "LHS 2001",
+ "pos_x": -54.0625,
+ "pos_y": 55.21875,
+ "pos_z": -67.25,
+ "stations": "Butler Installation,Asire Landing"
+ },
+ {
+ "name": "LHS 2018",
+ "pos_x": -25.28125,
+ "pos_y": 41.8125,
+ "pos_z": -53.5625,
+ "stations": "Vernadsky Dock"
+ },
+ {
+ "name": "LHS 2022",
+ "pos_x": 10.53125,
+ "pos_y": 40.25,
+ "pos_z": -58.5625,
+ "stations": "Whitworth Ring,Henricks City,Fabian Port,Forstchen Horizons,Shriver Point"
+ },
+ {
+ "name": "LHS 2026",
+ "pos_x": 43.34375,
+ "pos_y": 23.5625,
+ "pos_z": -41.09375,
+ "stations": "Sturckow Plant,Sharma Installation"
+ },
+ {
+ "name": "LHS 2029",
+ "pos_x": 27.40625,
+ "pos_y": 31.5,
+ "pos_z": -45.625,
+ "stations": "Spring Relay,Skvortsov Landing,McKay Legacy"
+ },
+ {
+ "name": "LHS 2037",
+ "pos_x": 33.78125,
+ "pos_y": 7.25,
+ "pos_z": -14.5,
+ "stations": "Tsibliyev Orbital,Meikle Dock,Bulgakov Point,McNair Port,Shepherd Lab"
+ },
+ {
+ "name": "LHS 205",
+ "pos_x": 34.03125,
+ "pos_y": -22.0625,
+ "pos_z": 12.625,
+ "stations": "Bunnell Oasis,Webb Refinery,Waldrop City"
+ },
+ {
+ "name": "LHS 2065",
+ "pos_x": 19.71875,
+ "pos_y": 11.8125,
+ "pos_z": -15.84375,
+ "stations": "Gubarev Vision"
+ },
+ {
+ "name": "LHS 2088",
+ "pos_x": -23.3125,
+ "pos_y": 25.71875,
+ "pos_z": -28.34375,
+ "stations": "Tanaka Terminal,Bacigalupi Ring,Timofeyevich Settlement,Baydukov Terminal,Comer Terminal"
+ },
+ {
+ "name": "LHS 2094",
+ "pos_x": 35,
+ "pos_y": 35,
+ "pos_z": -40,
+ "stations": "Yourkevitch Orbital,Malaspina Holdings,Patterson Vision,Kennicott Prospect,Shapiro Orbital"
+ },
+ {
+ "name": "LHS 21",
+ "pos_x": -18.21875,
+ "pos_y": -12.78125,
+ "pos_z": -55.375,
+ "stations": "Quimper Ring,Crook Orbital,Godwin Survey,Brunner Landing"
+ },
+ {
+ "name": "LHS 2106",
+ "pos_x": 35.65625,
+ "pos_y": 11.4375,
+ "pos_z": -13.34375,
+ "stations": "Gooch Vision"
+ },
+ {
+ "name": "LHS 2123",
+ "pos_x": -56.53125,
+ "pos_y": 42.40625,
+ "pos_z": -44.75,
+ "stations": "Oppenheimer Dock,Divis City,Swanson Orbital"
+ },
+ {
+ "name": "LHS 214",
+ "pos_x": -34.75,
+ "pos_y": 23.09375,
+ "pos_z": -51,
+ "stations": "Laird Vision"
+ },
+ {
+ "name": "LHS 215",
+ "pos_x": -20.59375,
+ "pos_y": 13.28125,
+ "pos_z": -18.3125,
+ "stations": "Spring Gateway,Feynman Gateway,Baker Depot,Laveykin Observatory,Diesel Orbital"
+ },
+ {
+ "name": "LHS 2150",
+ "pos_x": 104.3125,
+ "pos_y": 5.375,
+ "pos_z": 3.5625,
+ "stations": "Reynolds Port,Walter Ring,Taine Installation,Onufrienko Orbital,Fisher's Claim,Volynov Landing,Harness Installation,Walker Laboratory"
+ },
+ {
+ "name": "LHS 2157",
+ "pos_x": 26.21875,
+ "pos_y": 11.15625,
+ "pos_z": -7.3125,
+ "stations": "Plexico Plant,Tuan Orbital,Reed Lab,Allen Platform,DeLucas Port"
+ },
+ {
+ "name": "LHS 2158",
+ "pos_x": 60.0625,
+ "pos_y": 43.53125,
+ "pos_z": -33.8125,
+ "stations": "Mendez Holdings,Zettel Barracks"
+ },
+ {
+ "name": "LHS 2165",
+ "pos_x": 113,
+ "pos_y": 19.625,
+ "pos_z": -4.71875,
+ "stations": "Miletus Hub,Dyr City,Cochrane Gateway,May Vision"
+ },
+ {
+ "name": "LHS 2166",
+ "pos_x": 30.5,
+ "pos_y": 4.65625,
+ "pos_z": -0.59375,
+ "stations": "Lind Landing,Veach Hangar"
+ },
+ {
+ "name": "LHS 22",
+ "pos_x": 28.5625,
+ "pos_y": -28.90625,
+ "pos_z": -3.53125,
+ "stations": "Freud Station,Marshall Depot,Muhammad Ibn Battuta Prospect,Davis Beacon,Glenn City"
+ },
+ {
+ "name": "LHS 220",
+ "pos_x": -0.90625,
+ "pos_y": 11.5,
+ "pos_z": -41.46875,
+ "stations": "Culpeper Colony,Clauss Vision"
+ },
+ {
+ "name": "LHS 2205",
+ "pos_x": -13.90625,
+ "pos_y": 65.3125,
+ "pos_z": -53.875,
+ "stations": "Kuhn Port,Mills Hangar,Betancourt Refinery"
+ },
+ {
+ "name": "LHS 221",
+ "pos_x": -13.25,
+ "pos_y": 14.25,
+ "pos_z": -28.71875,
+ "stations": "Reynolds Terminal,Schiltberger Port,Lee Terminal,Mackenzie Refinery"
+ },
+ {
+ "name": "LHS 2212",
+ "pos_x": 16.53125,
+ "pos_y": 40.4375,
+ "pos_z": -29.3125,
+ "stations": "Heng Port,Veblen Prospect,Halsell Settlement"
+ },
+ {
+ "name": "LHS 2217",
+ "pos_x": 40.1875,
+ "pos_y": 50,
+ "pos_z": -30.15625,
+ "stations": "Selye Stop,Cantor Beacon"
+ },
+ {
+ "name": "LHS 223",
+ "pos_x": 7,
+ "pos_y": 4.5625,
+ "pos_z": -23.90625,
+ "stations": "Boole Landing,Wundt Dock"
+ },
+ {
+ "name": "LHS 2233",
+ "pos_x": 69.34375,
+ "pos_y": 21.03125,
+ "pos_z": 0.25,
+ "stations": "RenenBellot Penal colony,Gagnan Orbital,Roosa Colony"
+ },
+ {
+ "name": "LHS 2237",
+ "pos_x": -15.09375,
+ "pos_y": 59.21875,
+ "pos_z": -44.03125,
+ "stations": "Stasheff Terminal,Bunnell Estate"
+ },
+ {
+ "name": "LHS 224",
+ "pos_x": -7.5625,
+ "pos_y": 11.71875,
+ "pos_z": -26.46875,
+ "stations": "Gulyaev Keep,Bunsen Platform,Bowersox Relay"
+ },
+ {
+ "name": "LHS 2259",
+ "pos_x": 30.375,
+ "pos_y": 25.1875,
+ "pos_z": -8.03125,
+ "stations": "England Terminal,Aksyonov Orbital,Yegorov Orbital,Ejeta Terminal,Chaudhary Station,Utley Point,Garriott Station,Alexander Legacy"
+ },
+ {
+ "name": "LHS 2260",
+ "pos_x": 12.875,
+ "pos_y": 46.625,
+ "pos_z": -26.75,
+ "stations": "McClintock Gateway,Rasch Settlement,Rushd Gateway,Barba City"
+ },
+ {
+ "name": "LHS 2263",
+ "pos_x": -11.1875,
+ "pos_y": 51.65625,
+ "pos_z": -36,
+ "stations": "Usachov Mines"
+ },
+ {
+ "name": "LHS 2265",
+ "pos_x": 72.90625,
+ "pos_y": 68.1875,
+ "pos_z": -23.3125,
+ "stations": "Abe Reserve,Krylov Horizons,McMullen's Inheritance,Forsskal Gateway"
+ },
+ {
+ "name": "LHS 2270",
+ "pos_x": 8.3125,
+ "pos_y": 53.59375,
+ "pos_z": -31.5,
+ "stations": "Santarem Refinery"
+ },
+ {
+ "name": "LHS 2274",
+ "pos_x": 8.21875,
+ "pos_y": 58.28125,
+ "pos_z": -33.9375,
+ "stations": "Wilson Dock,Mitchell Landing,Borisenko Relay"
+ },
+ {
+ "name": "LHS 229",
+ "pos_x": -5.90625,
+ "pos_y": 16.625,
+ "pos_z": -33.34375,
+ "stations": "Archimedes Prospect,Thagard Dock"
+ },
+ {
+ "name": "LHS 2310",
+ "pos_x": 43.75,
+ "pos_y": -16,
+ "pos_z": 22.5625,
+ "stations": "Herbert Market,Levy Hub,Tsunenaga Arena,McCaffrey Terminal,Noriega Settlement,Rorschach's Progress,MacLeod Station,Waldrop Gateway,Chadwick Hub,Valdes Terminal,Fisher Lab,Fuchs Hub,Narbeth Enterprise,White Ring,Flynn Legacy,Jakes Hub"
+ },
+ {
+ "name": "LHS 2317",
+ "pos_x": 7.625,
+ "pos_y": 69.34375,
+ "pos_z": -34.125,
+ "stations": "Cartan Platform,Cook Refinery"
+ },
+ {
+ "name": "LHS 2335",
+ "pos_x": 52.09375,
+ "pos_y": 25.34375,
+ "pos_z": 5.6875,
+ "stations": "Haberlandt Hangar,Borisenko Base,Dunn Dock,Bykovsky Plant,Montrose Bastion"
+ },
+ {
+ "name": "LHS 2337",
+ "pos_x": 9.9375,
+ "pos_y": 66.25,
+ "pos_z": -28.90625,
+ "stations": "Malocello Terminal,Clayton Works"
+ },
+ {
+ "name": "LHS 235",
+ "pos_x": 23.5,
+ "pos_y": 1.25,
+ "pos_z": -17.125,
+ "stations": "Pauling Port,Shatalov Gateway,Rondon Installation"
+ },
+ {
+ "name": "LHS 2363",
+ "pos_x": -11.9375,
+ "pos_y": 62.25,
+ "pos_z": -31.78125,
+ "stations": "Pontes Installation,Midgley Landing"
+ },
+ {
+ "name": "LHS 2370",
+ "pos_x": 52.6875,
+ "pos_y": 46.5625,
+ "pos_z": 0.78125,
+ "stations": "Creighton Gateway,Moran Relay,Molina Terminal,Bulmer Vision,Leavitt Hub"
+ },
+ {
+ "name": "LHS 2405",
+ "pos_x": -45.875,
+ "pos_y": 45.5625,
+ "pos_z": -36.28125,
+ "stations": "Willis Beacon,Godwin City,Kidman Ring,Hewish Beacon"
+ },
+ {
+ "name": "LHS 2412",
+ "pos_x": 55.09375,
+ "pos_y": 62.28125,
+ "pos_z": 1.875,
+ "stations": "Hauck Terminal,Hopkins Hub,Ramanujan Enterprise,Foden Survey"
+ },
+ {
+ "name": "LHS 2429",
+ "pos_x": 24.71875,
+ "pos_y": 13.375,
+ "pos_z": 6.875,
+ "stations": "Acaba Dock,Antonelli City,Sucharitkul Settlement,Bakewell Station,Goeppert-Mayer Depot,Marshall's Claim"
+ },
+ {
+ "name": "LHS 2430",
+ "pos_x": -2.8125,
+ "pos_y": 52.03125,
+ "pos_z": -18.46875,
+ "stations": "Dana City,Hahn Dock,Harvey Station,de Andrade Horizons,Ellern Base"
+ },
+ {
+ "name": "LHS 2441",
+ "pos_x": 36.3125,
+ "pos_y": 11.5,
+ "pos_z": 13.53125,
+ "stations": "Asimov Landing,Mayr Relay"
+ },
+ {
+ "name": "LHS 2459",
+ "pos_x": -11,
+ "pos_y": 10.78125,
+ "pos_z": -8.21875,
+ "stations": "Robinson Prospect"
+ },
+ {
+ "name": "LHS 246",
+ "pos_x": -19,
+ "pos_y": 22.5625,
+ "pos_z": -28.21875,
+ "stations": "Gooch Terminal,Bacon Terminal,Winne City,Melvill Vision,Darnielle's Progress"
+ },
+ {
+ "name": "LHS 2471",
+ "pos_x": 18.96875,
+ "pos_y": 42.09375,
+ "pos_z": -1.3125,
+ "stations": "Michelson Gateway,Teng-hui Dock,Baird City,Belyayev Terminal,Fossey Port,Artsebarsky Enterprise,Precourt Hub,Reisman's Inheritance,Ito Laboratory,Sturt Point,Dowling Holdings"
+ },
+ {
+ "name": "LHS 2477",
+ "pos_x": 67.8125,
+ "pos_y": 31.1875,
+ "pos_z": 26.9375,
+ "stations": "Fuglesang Base,Mach Vision,Phillips Mines"
+ },
+ {
+ "name": "LHS 2482",
+ "pos_x": 22.75,
+ "pos_y": 63.90625,
+ "pos_z": -3.71875,
+ "stations": "Yourkevitch Terminal,Bacigalupi Terminal,Froude Hub"
+ },
+ {
+ "name": "LHS 2494",
+ "pos_x": 35.53125,
+ "pos_y": 59.03125,
+ "pos_z": 5.09375,
+ "stations": "Ivanishin City,Ericsson Orbital,Patsayev Holdings,McClintock Enterprise,Patrick Enterprise"
+ },
+ {
+ "name": "LHS 250",
+ "pos_x": -19.1875,
+ "pos_y": 24.34375,
+ "pos_z": -29.53125,
+ "stations": "Griffith Prospect,Iqbal Works,Kondakova Hangar,Noguchi Dock,Mayer Landing"
+ },
+ {
+ "name": "LHS 2524",
+ "pos_x": 57.59375,
+ "pos_y": 96.6875,
+ "pos_z": 15.9375,
+ "stations": "Haberlandt Station,Thiele Station,Rozhdestvensky Hub"
+ },
+ {
+ "name": "LHS 2541",
+ "pos_x": 29.8125,
+ "pos_y": 63.96875,
+ "pos_z": 6.625,
+ "stations": "Miletus Dock,Kroehl Plant,Hannu Base,Moore Installation,Bokeili Hub"
+ },
+ {
+ "name": "LHS 259",
+ "pos_x": -7.625,
+ "pos_y": 42.28125,
+ "pos_z": -47.90625,
+ "stations": "Linaweaver Enterprise,Anthony Orbital,Bakewell Observatory,Garan Laboratory"
+ },
+ {
+ "name": "LHS 262",
+ "pos_x": -6.90625,
+ "pos_y": 22.875,
+ "pos_z": -23.8125,
+ "stations": "Boodt Port"
+ },
+ {
+ "name": "LHS 263",
+ "pos_x": 23.0625,
+ "pos_y": -8.875,
+ "pos_z": 9.5625,
+ "stations": "Pausch Enterprise,Laval Hub,Wilcutt Orbital"
+ },
+ {
+ "name": "LHS 2637",
+ "pos_x": -22.5625,
+ "pos_y": 74.6875,
+ "pos_z": -15.53125,
+ "stations": "Perez Ring,Bujold Enterprise,Ledyard Keep,Arnarson Enterprise"
+ },
+ {
+ "name": "LHS 2651",
+ "pos_x": -22.84375,
+ "pos_y": 60.375,
+ "pos_z": -13.8125,
+ "stations": "Kennan Dock,Bergerac Ring,Shipton Orbital,Pelt Arsenal"
+ },
+ {
+ "name": "LHS 266",
+ "pos_x": 17.375,
+ "pos_y": 46.3125,
+ "pos_z": -45.96875,
+ "stations": "Adamson Beacon,Mukai Horizons,Musa al-Khwarizmi Market,Armstrong Keep,Jun Station"
+ },
+ {
+ "name": "LHS 2687",
+ "pos_x": -61.15625,
+ "pos_y": 83.3125,
+ "pos_z": -35.0625,
+ "stations": "Williamson Hub,Garnier City,Heyerdahl Port,Narita Gateway,Crichton Settlement,Wells Terminal,Jenkinson City,Haarsma Station,Morrison Depot"
+ },
+ {
+ "name": "LHS 2691",
+ "pos_x": -4.1875,
+ "pos_y": 65,
+ "pos_z": 3.21875,
+ "stations": "Arnason Plant"
+ },
+ {
+ "name": "LHS 2725",
+ "pos_x": 19.125,
+ "pos_y": 67.46875,
+ "pos_z": 23.96875,
+ "stations": "Banks Survey,Merril Station,Platt Installation"
+ },
+ {
+ "name": "LHS 274",
+ "pos_x": 35.8125,
+ "pos_y": 23.28125,
+ "pos_z": -13.25,
+ "stations": "Davidson Holdings,Freud Dock,White Terminal,Teller Port,Merchiston Vista,Al Saud Ring,McCoy Enterprise,Carrier Enterprise,Cavendish City,Soddy Terminal,Chamitoff Station,Edgeworth Park,Swanwick's Progress,Gerlache Hub,Linnehan Base,Kippax Base"
+ },
+ {
+ "name": "LHS 2764a",
+ "pos_x": -4.71875,
+ "pos_y": 54.125,
+ "pos_z": 0.65625,
+ "stations": "Low Orbital,Galouye Hub"
+ },
+ {
+ "name": "LHS 277",
+ "pos_x": 49.84375,
+ "pos_y": 17.875,
+ "pos_z": -4.15625,
+ "stations": "Lee Settlement,Waldeck Prospect,Rowley Port,Taylor Port,Aikin Relay"
+ },
+ {
+ "name": "LHS 2771",
+ "pos_x": -5.78125,
+ "pos_y": 64.09375,
+ "pos_z": 10.5625,
+ "stations": "Sarafanov Vision,Pudwill Gorie Hub,Cayley Dock"
+ },
+ {
+ "name": "LHS 278",
+ "pos_x": -5.90625,
+ "pos_y": 38.65625,
+ "pos_z": -29.90625,
+ "stations": "Fossum Ring,Jahn's Folly,Maudslay Station,Broglie Gateway,Effinger Survey,Tyson Vision,Przhevalsky Base"
+ },
+ {
+ "name": "LHS 2789",
+ "pos_x": -34.34375,
+ "pos_y": 71,
+ "pos_z": -8.78125,
+ "stations": "Crook Settlement,Krupkat Relay,Leichhardt Vision"
+ },
+ {
+ "name": "LHS 28",
+ "pos_x": -18.0625,
+ "pos_y": 5.8125,
+ "pos_z": -40.1875,
+ "stations": "Flade Enterprise,Hedley City,Holland Base,Payne Arsenal,Berezovoy Gateway"
+ },
+ {
+ "name": "LHS 2813",
+ "pos_x": 48.0625,
+ "pos_y": 9.40625,
+ "pos_z": 43.0625,
+ "stations": "Celsius Park"
+ },
+ {
+ "name": "LHS 2819",
+ "pos_x": -30.5,
+ "pos_y": 38.5625,
+ "pos_z": -13.4375,
+ "stations": "Tasaki Freeport,Pook Port"
+ },
+ {
+ "name": "LHS 283",
+ "pos_x": -20.78125,
+ "pos_y": 29.59375,
+ "pos_z": -23.53125,
+ "stations": "Khan Port"
+ },
+ {
+ "name": "LHS 287",
+ "pos_x": 1.03125,
+ "pos_y": 29.59375,
+ "pos_z": -16.5,
+ "stations": "Werner von Siemens Port,Fettman Base"
+ },
+ {
+ "name": "LHS 2875",
+ "pos_x": 14.71875,
+ "pos_y": 68.21875,
+ "pos_z": 43.3125,
+ "stations": "Kerwin Horizons,Hoffman Vision,Lyell City"
+ },
+ {
+ "name": "LHS 2884",
+ "pos_x": -22,
+ "pos_y": 48.40625,
+ "pos_z": 1.78125,
+ "stations": "Abnett Platform,Chios Landing,Sawyer City,Kennedy Vision"
+ },
+ {
+ "name": "LHS 2887",
+ "pos_x": -7.34375,
+ "pos_y": 26.78125,
+ "pos_z": 5.71875,
+ "stations": "Massimino Dock,Musabayev Enterprise,Ochoa Installation"
+ },
+ {
+ "name": "LHS 289",
+ "pos_x": 53.5625,
+ "pos_y": 37.1875,
+ "pos_z": -2.90625,
+ "stations": "Crick Outpost,Lee Camp"
+ },
+ {
+ "name": "LHS 2925",
+ "pos_x": -17.1875,
+ "pos_y": 60.5625,
+ "pos_z": 17.03125,
+ "stations": "Hillary Co-operative,Houtman Works,Le Guin Penal colony,Betancourt Orbital,Brooks Hub"
+ },
+ {
+ "name": "LHS 293",
+ "pos_x": 1.84375,
+ "pos_y": 28.3125,
+ "pos_z": -14.4375,
+ "stations": "Verne Settlement"
+ },
+ {
+ "name": "LHS 2931",
+ "pos_x": -25.09375,
+ "pos_y": 68.15625,
+ "pos_z": 14.625,
+ "stations": "Lee Station,Schmitz Survey"
+ },
+ {
+ "name": "LHS 2936",
+ "pos_x": -31.6875,
+ "pos_y": 55.5625,
+ "pos_z": 0.5,
+ "stations": "Fraser Orbital,Zebrowski Orbital,Kessel Port,Furrer Vista,Bixby's Progress"
+ },
+ {
+ "name": "LHS 2948",
+ "pos_x": -21.6875,
+ "pos_y": 60.4375,
+ "pos_z": 15.09375,
+ "stations": "Higginbotham Port,Balandin Prospect,Liska Port,McArthur Installation"
+ },
+ {
+ "name": "LHS 295",
+ "pos_x": 15.71875,
+ "pos_y": 33.46875,
+ "pos_z": -12.21875,
+ "stations": "Carlisle Dock,Mitchell Prospect"
+ },
+ {
+ "name": "LHS 2983",
+ "pos_x": -3.03125,
+ "pos_y": 80.15625,
+ "pos_z": 56.8125,
+ "stations": "Schade Holdings,Niven Landing,Heyerdahl Base"
+ },
+ {
+ "name": "LHS 2995",
+ "pos_x": -5.9375,
+ "pos_y": 50.96875,
+ "pos_z": 32.0625,
+ "stations": "Schmitt Station,Coulomb Ring,Murray Station"
+ },
+ {
+ "name": "LHS 3005",
+ "pos_x": -12.15625,
+ "pos_y": 67.84375,
+ "pos_z": 40.0625,
+ "stations": "Cartmill City,Timofeyevich Arsenal,Walker Relay"
+ },
+ {
+ "name": "LHS 3006",
+ "pos_x": -21.96875,
+ "pos_y": 29.09375,
+ "pos_z": -1.71875,
+ "stations": "Asprin Palace,Leonard Nimoy Station,Frimout Legacy,Hodgkin Vision"
+ },
+ {
+ "name": "LHS 301",
+ "pos_x": 28.84375,
+ "pos_y": 52.34375,
+ "pos_z": -8.625,
+ "stations": "McArthur Orbital,Payette Dock,O'Connor Terminal,Poindexter Prospect,Bramah Forum,Wiener's Progress"
+ },
+ {
+ "name": "LHS 302",
+ "pos_x": 9.96875,
+ "pos_y": 53.1875,
+ "pos_z": -16.3125,
+ "stations": "Chapman Orbital,Kavandi Dock"
+ },
+ {
+ "name": "LHS 3022",
+ "pos_x": 19.15625,
+ "pos_y": 35.1875,
+ "pos_z": 53.125,
+ "stations": "Rothfuss Freeport"
+ },
+ {
+ "name": "LHS 3026",
+ "pos_x": -31.625,
+ "pos_y": 89.78125,
+ "pos_z": 45.375,
+ "stations": "Doctorow Beacon,Barth Horizons"
+ },
+ {
+ "name": "LHS 304",
+ "pos_x": 10.84375,
+ "pos_y": 41.125,
+ "pos_z": -11.09375,
+ "stations": "Love Colony,Andrews Silo"
+ },
+ {
+ "name": "LHS 306",
+ "pos_x": 30.09375,
+ "pos_y": 28.84375,
+ "pos_z": 2.96875,
+ "stations": "Melvin Port,Tokarev Orbital,Landsteiner Dock"
+ },
+ {
+ "name": "LHS 3067",
+ "pos_x": -4.625,
+ "pos_y": 59.46875,
+ "pos_z": 59.375,
+ "stations": "Glenn Station,Galiano Point,Jun Laboratory,Borisenko Exchange,Revin Platform"
+ },
+ {
+ "name": "LHS 3079",
+ "pos_x": 21.78125,
+ "pos_y": 30.40625,
+ "pos_z": 65.96875,
+ "stations": "Ross City,Borisenko Point,Still Port,Watson Orbital,Gloss Vision"
+ },
+ {
+ "name": "LHS 3080",
+ "pos_x": -21.625,
+ "pos_y": 42.90625,
+ "pos_z": 21.375,
+ "stations": "Buckey Installation,Aubakirov Colony"
+ },
+ {
+ "name": "LHS 3097",
+ "pos_x": 20.28125,
+ "pos_y": 46.125,
+ "pos_z": 90.625,
+ "stations": "Wallace City,Wyndham Depot,Virtanen Gateway,Singer Ring"
+ },
+ {
+ "name": "LHS 3117",
+ "pos_x": 1.71875,
+ "pos_y": 26.28125,
+ "pos_z": 41.59375,
+ "stations": "Lyakhov Terminal"
+ },
+ {
+ "name": "LHS 3119",
+ "pos_x": 42,
+ "pos_y": -3.6875,
+ "pos_z": 58.9375,
+ "stations": "Yang Refinery,Novitski Platform,Reed Observatory,Cartmill Terminal"
+ },
+ {
+ "name": "LHS 3142",
+ "pos_x": -26.65625,
+ "pos_y": 57.3125,
+ "pos_z": 51.5,
+ "stations": "Clark Orbital,Underwood Orbital"
+ },
+ {
+ "name": "LHS 3163",
+ "pos_x": -20.125,
+ "pos_y": 39.3125,
+ "pos_z": 39.71875,
+ "stations": "Malenchenko Landing,Butler Penal colony,Kovalyonok Hangar"
+ },
+ {
+ "name": "LHS 3167",
+ "pos_x": 29.9375,
+ "pos_y": -11.03125,
+ "pos_z": 33.9375,
+ "stations": "Volta Market,Volynov Ring,Cartwright Station,Smith Terminal,McMonagle Station"
+ },
+ {
+ "name": "LHS 317",
+ "pos_x": 44.65625,
+ "pos_y": 27.03125,
+ "pos_z": 15.03125,
+ "stations": "Northrop Port,Boas Enterprise,Crown City,Jun Terminal,Zoline Depot"
+ },
+ {
+ "name": "LHS 3182",
+ "pos_x": 35.65625,
+ "pos_y": 1.1875,
+ "pos_z": 76.71875,
+ "stations": "Carver Orbital,Shepherd Bastion,Cenker City,Buckland Terminal,Landsteiner Depot"
+ },
+ {
+ "name": "LHS 3197",
+ "pos_x": 0.75,
+ "pos_y": 14.15625,
+ "pos_z": 36.125,
+ "stations": "Fullerton Enterprise,Szilard Orbital,Aguirre Hub"
+ },
+ {
+ "name": "LHS 3246",
+ "pos_x": -14.5625,
+ "pos_y": 27.625,
+ "pos_z": 60.34375,
+ "stations": "Hertz Hangar,Evans Terminal"
+ },
+ {
+ "name": "LHS 3262",
+ "pos_x": -24.125,
+ "pos_y": 18.84375,
+ "pos_z": 4.90625,
+ "stations": "Lacaille Prospect,Sy Base,Whitworth Park,Jacquard Hub,Haber Installation"
+ },
+ {
+ "name": "LHS 3295",
+ "pos_x": 27.03125,
+ "pos_y": -15.96875,
+ "pos_z": 25.1875,
+ "stations": "Yang Hub,Penn's Progress,Matteucci Port,Krusvar's Claim,Richards City"
+ },
+ {
+ "name": "LHS 331",
+ "pos_x": -24.40625,
+ "pos_y": 63.4375,
+ "pos_z": -20.65625,
+ "stations": "Dukaj Legacy,Crowley Hub,Dyr Enterprise,Asaro Gateway,Maire Orbital,Gold Station,Gentle Enterprise,Verrazzano Keep,Sargent Orbital,Loncke Ring"
+ },
+ {
+ "name": "LHS 3315",
+ "pos_x": -21.65625,
+ "pos_y": 14.5,
+ "pos_z": 71.03125,
+ "stations": "Wittgenstein Hangar,Hooke Station,Precourt Relay"
+ },
+ {
+ "name": "LHS 3317",
+ "pos_x": 11.0625,
+ "pos_y": -6.125,
+ "pos_z": 59.03125,
+ "stations": "Savinykh Orbital,Gagarin Dock,Lyell Port,Scheutz Orbital,Smith Hub"
+ },
+ {
+ "name": "LHS 332",
+ "pos_x": 43.1875,
+ "pos_y": 5.875,
+ "pos_z": 24.78125,
+ "stations": "Scithers Penal colony,Pelt Penal colony"
+ },
+ {
+ "name": "LHS 3320",
+ "pos_x": -12.125,
+ "pos_y": 8.28125,
+ "pos_z": 79.78125,
+ "stations": "Jett Hub"
+ },
+ {
+ "name": "LHS 3333",
+ "pos_x": -48.21875,
+ "pos_y": 27.875,
+ "pos_z": 42.40625,
+ "stations": "Anderson Terminal"
+ },
+ {
+ "name": "LHS 3343",
+ "pos_x": -38.9375,
+ "pos_y": 21.5625,
+ "pos_z": 11.4375,
+ "stations": "Johnson Refinery"
+ },
+ {
+ "name": "LHS 3356",
+ "pos_x": -10.6875,
+ "pos_y": 3.625,
+ "pos_z": 22.65625,
+ "stations": "Moffitt Hub,Bentham Dock,Lewis Dock,Blackman Ring,Pytheas Orbital,McDonald Lab,Wellman Beacon,Dobzhansky Silo"
+ },
+ {
+ "name": "LHS 336",
+ "pos_x": 26.5625,
+ "pos_y": 5.78125,
+ "pos_z": 16.125,
+ "stations": "Klimuk Station,Doi Enterprise,van Vogt Silo,Payette Gateway"
+ },
+ {
+ "name": "LHS 3384",
+ "pos_x": -20.34375,
+ "pos_y": -0.03125,
+ "pos_z": 61.96875,
+ "stations": "Michelson Gateway,Avdeyev Platform,Cavendish Mines"
+ },
+ {
+ "name": "LHS 3385",
+ "pos_x": -69.21875,
+ "pos_y": 28.8125,
+ "pos_z": 30.25,
+ "stations": "Rasch Plant,Scalzi Station,Robinson Survey"
+ },
+ {
+ "name": "LHS 3388",
+ "pos_x": -72.9375,
+ "pos_y": 18.59375,
+ "pos_z": 92.9375,
+ "stations": "Hauck Port,Kiernan Penal colony,Howard Prospect"
+ },
+ {
+ "name": "LHS 340",
+ "pos_x": 20.46875,
+ "pos_y": 8.25,
+ "pos_z": 12.5,
+ "stations": "Khan Holdings"
+ },
+ {
+ "name": "LHS 3421",
+ "pos_x": 27.5625,
+ "pos_y": -33.375,
+ "pos_z": 72.5625,
+ "stations": "McMahon Mines"
+ },
+ {
+ "name": "LHS 3437",
+ "pos_x": -25.25,
+ "pos_y": -12.75,
+ "pos_z": 63.53125,
+ "stations": "Gardner Stop"
+ },
+ {
+ "name": "LHS 3439",
+ "pos_x": 10.21875,
+ "pos_y": -26.53125,
+ "pos_z": 60.8125,
+ "stations": "Tenn Mine,Gibson Mines,Lucretius Base,Clute Enterprise"
+ },
+ {
+ "name": "LHS 3447",
+ "pos_x": -43.1875,
+ "pos_y": -5.28125,
+ "pos_z": 56.15625,
+ "stations": "Trevithick Dock,Bluford Orbital,Lawson Orbital,Worlidge Terminal,Oleskiw Station,Dalton Gateway,Fairbairn Station,Yaping Enterprise,Avdeyev Laboratory,Leinster Survey,la Cosa Base"
+ },
+ {
+ "name": "LHS 3450",
+ "pos_x": -108.9375,
+ "pos_y": 25.46875,
+ "pos_z": 32.0625,
+ "stations": "Tombaugh Survey,Gorbatko Landing"
+ },
+ {
+ "name": "LHS 346",
+ "pos_x": 38.96875,
+ "pos_y": 20.21875,
+ "pos_z": 28.96875,
+ "stations": "McArthur Gateway,Morukov Orbital,Svavarsson Installation"
+ },
+ {
+ "name": "LHS 3479",
+ "pos_x": -50,
+ "pos_y": -12.9375,
+ "pos_z": 56.28125,
+ "stations": "Pavlou Depot,Blenkinsop Landing,Massimino Terminal"
+ },
+ {
+ "name": "LHS 350",
+ "pos_x": -1.46875,
+ "pos_y": 44.59375,
+ "pos_z": 5.75,
+ "stations": "Shumil Base,al-Haytham Enterprise,Oltion Dock"
+ },
+ {
+ "name": "LHS 3505",
+ "pos_x": -33.96875,
+ "pos_y": -12.40625,
+ "pos_z": 36.9375,
+ "stations": "Oleskiw Survey,Olivas City,Helmholtz Port,Hewish Station"
+ },
+ {
+ "name": "LHS 351",
+ "pos_x": 8.40625,
+ "pos_y": 59.875,
+ "pos_z": 17.5625,
+ "stations": "Tapinas Relay,Heck Survey"
+ },
+ {
+ "name": "LHS 3516",
+ "pos_x": -9.71875,
+ "pos_y": -31.03125,
+ "pos_z": 56.53125,
+ "stations": "Allen Settlement"
+ },
+ {
+ "name": "LHS 353",
+ "pos_x": 19.125,
+ "pos_y": 38.625,
+ "pos_z": 21.96875,
+ "stations": "Morgan Landing"
+ },
+ {
+ "name": "LHS 3531",
+ "pos_x": 1.4375,
+ "pos_y": -11.1875,
+ "pos_z": 16.78125,
+ "stations": "Mining Station 1,Jones Landing"
+ },
+ {
+ "name": "LHS 3549",
+ "pos_x": -29.625,
+ "pos_y": 6.125,
+ "pos_z": -1.9375,
+ "stations": "Cenker Orbital,Carey Station,Weston Refinery,Dunn Works,Volk Installation,Alvarado Prospect"
+ },
+ {
+ "name": "LHS 355",
+ "pos_x": 1.09375,
+ "pos_y": 46.15625,
+ "pos_z": 9.71875,
+ "stations": "Ramelli City,McIntyre Prospect,Tito Enterprise,Crown Terminal,Steinmuller Holdings"
+ },
+ {
+ "name": "LHS 3564",
+ "pos_x": -61.5625,
+ "pos_y": -32.25,
+ "pos_z": 53.46875,
+ "stations": "Froude Enterprise,Maine Hub,Lee Hub,Siemens Settlement"
+ },
+ {
+ "name": "LHS 3577",
+ "pos_x": -40.59375,
+ "pos_y": 0.625,
+ "pos_z": 3.96875,
+ "stations": "Dorsey Ring,Elgin Terminal,Gernsback Hub"
+ },
+ {
+ "name": "LHS 3586",
+ "pos_x": -82.09375,
+ "pos_y": 25.96875,
+ "pos_z": -23.15625,
+ "stations": "Novitski Survey"
+ },
+ {
+ "name": "LHS 3589",
+ "pos_x": -60.40625,
+ "pos_y": -12.5,
+ "pos_z": 21.25,
+ "stations": "Roosa Gateway,Appel Orbital,Ivanov Terminal,Cleave Point,Gibson Enterprise"
+ },
+ {
+ "name": "LHS 3593",
+ "pos_x": -35.65625,
+ "pos_y": -16.28125,
+ "pos_z": 22.46875,
+ "stations": "Piercy's Folly,Born Hub,Shelley Base,Howe City,Tombaugh Port"
+ },
+ {
+ "name": "LHS 3595",
+ "pos_x": -22.3125,
+ "pos_y": 4.40625,
+ "pos_z": -3.53125,
+ "stations": "Chaudhary Hub"
+ },
+ {
+ "name": "LHS 3598",
+ "pos_x": -59.84375,
+ "pos_y": -25.40625,
+ "pos_z": 33.5,
+ "stations": "Carleson Camp,Jemison Colony"
+ },
+ {
+ "name": "LHS 3602",
+ "pos_x": -16.84375,
+ "pos_y": 41.53125,
+ "pos_z": 52.96875,
+ "stations": "Otto Station,Shukor Terminal"
+ },
+ {
+ "name": "LHS 3615",
+ "pos_x": 8.65625,
+ "pos_y": -37.78125,
+ "pos_z": 42.09375,
+ "stations": "Wrangell Port"
+ },
+ {
+ "name": "LHS 3631",
+ "pos_x": -91.625,
+ "pos_y": 30.34375,
+ "pos_z": -31.65625,
+ "stations": "Burbank Hub,Dyson Laboratory,Almagro Relay"
+ },
+ {
+ "name": "LHS 3666",
+ "pos_x": 6.96875,
+ "pos_y": -59,
+ "pos_z": 56.96875,
+ "stations": "Meyrink Orbital,Atiyah Orbital,Szameit Port,Eisenstein Base"
+ },
+ {
+ "name": "LHS 3675",
+ "pos_x": 18.53125,
+ "pos_y": -52.28125,
+ "pos_z": 50.5,
+ "stations": "Broderick Gateway,Kelly Market"
+ },
+ {
+ "name": "LHS 3677",
+ "pos_x": -60.9375,
+ "pos_y": -40.375,
+ "pos_z": 34.75,
+ "stations": "Clifford Terminal"
+ },
+ {
+ "name": "LHS 369",
+ "pos_x": 9.71875,
+ "pos_y": 26.34375,
+ "pos_z": 22.15625,
+ "stations": "Dunyach's Folly,Young Silo,Zillig Dock"
+ },
+ {
+ "name": "LHS 3705",
+ "pos_x": -85.65625,
+ "pos_y": -10.375,
+ "pos_z": -2.625,
+ "stations": "Antonio de Andrade Settlement,Ricci Installation,Vries Arsenal"
+ },
+ {
+ "name": "LHS 371",
+ "pos_x": -9.625,
+ "pos_y": 49.4375,
+ "pos_z": 17.625,
+ "stations": "Cavendish Station,Tani Enterprise,Nusslein-Volhard Station,Pawelczyk Orbital"
+ },
+ {
+ "name": "LHS 3713",
+ "pos_x": -55.96875,
+ "pos_y": -20.15625,
+ "pos_z": 9.125,
+ "stations": "Binnie Enterprise,Greenleaf Prospect,Ashby Dock,Gillekens Orbital,Nielsen Installation"
+ },
+ {
+ "name": "LHS 3728",
+ "pos_x": 3.3125,
+ "pos_y": -61.71875,
+ "pos_z": 50.09375,
+ "stations": "Lilienthal City,Maskelyne Settlement"
+ },
+ {
+ "name": "LHS 3739",
+ "pos_x": -11.53125,
+ "pos_y": -61.5625,
+ "pos_z": 45.90625,
+ "stations": "Aleksandrov Ring,Gutierrez City,Fancher Survey,Henry Enterprise,Cady Observatory"
+ },
+ {
+ "name": "LHS 374",
+ "pos_x": 12.46875,
+ "pos_y": 40.0625,
+ "pos_z": 36.15625,
+ "stations": "Harness Landing,Kandel Terminal,Gold Colony"
+ },
+ {
+ "name": "LHS 3746",
+ "pos_x": -2.21875,
+ "pos_y": -26.125,
+ "pos_z": 19.40625,
+ "stations": "Gamow Terminal,Readdy Base,Reisman Hangar"
+ },
+ {
+ "name": "LHS 3749",
+ "pos_x": -69.5625,
+ "pos_y": 12.46875,
+ "pos_z": -22.4375,
+ "stations": "Roddenberry Orbital,Elgin Arsenal"
+ },
+ {
+ "name": "LHS 3756",
+ "pos_x": -43.09375,
+ "pos_y": -50.625,
+ "pos_z": 3.46875,
+ "stations": "Bruce Prospect,Jones Settlement"
+ },
+ {
+ "name": "LHS 3767",
+ "pos_x": -99.90625,
+ "pos_y": -51.1875,
+ "pos_z": 14.4375,
+ "stations": "Schachner Terminal,Jones Dock"
+ },
+ {
+ "name": "LHS 3776",
+ "pos_x": -13.09375,
+ "pos_y": -27.03125,
+ "pos_z": 16.0625,
+ "stations": "Aguirre Terminal,Blackman Landing,Thompson Silo"
+ },
+ {
+ "name": "LHS 378",
+ "pos_x": 13.90625,
+ "pos_y": 28.46875,
+ "pos_z": 35.03125,
+ "stations": "McCoy Platform,Tuan Survey,Ericsson Beacon"
+ },
+ {
+ "name": "LHS 3781",
+ "pos_x": -60.03125,
+ "pos_y": -26.125,
+ "pos_z": 4.15625,
+ "stations": "Grabe Resort,Lawson Prospect,Aubakirov Colony"
+ },
+ {
+ "name": "LHS 380",
+ "pos_x": 5.28125,
+ "pos_y": 6.75,
+ "pos_z": 10.875,
+ "stations": "Qureshi Orbital,Ray Enterprise,Mastracchio Enterprise"
+ },
+ {
+ "name": "LHS 3802",
+ "pos_x": 17.65625,
+ "pos_y": -48.34375,
+ "pos_z": 35.375,
+ "stations": "White Ring,Tokubei Terminal,Sterling Vista,Henry Enterprise"
+ },
+ {
+ "name": "LHS 3804",
+ "pos_x": 5.03125,
+ "pos_y": -31.75,
+ "pos_z": 21.78125,
+ "stations": "Sharman Point,Kuhn Dock"
+ },
+ {
+ "name": "LHS 3836",
+ "pos_x": 9.46875,
+ "pos_y": -16,
+ "pos_z": 12.09375,
+ "stations": "Gell-Mann Station,Faraday Settlement,Olsen Bastion,Khrenov Relay,Wakata City"
+ },
+ {
+ "name": "LHS 3872",
+ "pos_x": -34.28125,
+ "pos_y": -53.3125,
+ "pos_z": 15.5,
+ "stations": "Curbeam Hub,Cugnot Terminal,McArthur Hub,Polyakov Enterprise,Sanger Survey"
+ },
+ {
+ "name": "LHS 3877",
+ "pos_x": -74.28125,
+ "pos_y": 10.90625,
+ "pos_z": -30.84375,
+ "stations": "Foreman City,Williams Port,Wang Ring,Nyberg Port,Macarthur Port,Shaw City,Gerst Ring,Gresley Gateway,Ejeta Port"
+ },
+ {
+ "name": "LHS 3885",
+ "pos_x": -7.03125,
+ "pos_y": -24.28125,
+ "pos_z": 8.90625,
+ "stations": "Alvarado Ring,Mohun City"
+ },
+ {
+ "name": "LHS 3899",
+ "pos_x": -32.25,
+ "pos_y": -40.09375,
+ "pos_z": 5.28125,
+ "stations": "Galindo Survey,Elcano Prospect"
+ },
+ {
+ "name": "LHS 391",
+ "pos_x": -15.90625,
+ "pos_y": 45.15625,
+ "pos_z": 21.375,
+ "stations": "Roddenberry Relay,Younghusband Orbital,Wilson Station,Kornbluth Port,Raleigh Holdings"
+ },
+ {
+ "name": "LHS 392",
+ "pos_x": 6.375,
+ "pos_y": 30.46875,
+ "pos_z": 36.46875,
+ "stations": "Binder Orbital,Carver Orbital,Reynolds Plant"
+ },
+ {
+ "name": "LHS 3921",
+ "pos_x": 42.53125,
+ "pos_y": -68.375,
+ "pos_z": 44.34375,
+ "stations": "Holdstock Market,Goonan Keep,Grijalva Hub"
+ },
+ {
+ "name": "LHS 3923",
+ "pos_x": -52.8125,
+ "pos_y": -20.90625,
+ "pos_z": -12.5,
+ "stations": "Kadenyuk Station,Chomsky Port,Maxwell Hub,Stebler Landing,Gunn Beacon"
+ },
+ {
+ "name": "LHS 3931",
+ "pos_x": -92.15625,
+ "pos_y": -53.75,
+ "pos_z": -17.125,
+ "stations": "Penn Ring,Anderson Ring"
+ },
+ {
+ "name": "LHS 3946",
+ "pos_x": -65.90625,
+ "pos_y": -38.90625,
+ "pos_z": -13.25,
+ "stations": "Weinbaum Station,Anthony Refinery"
+ },
+ {
+ "name": "LHS 3947",
+ "pos_x": -50.25,
+ "pos_y": -56.875,
+ "pos_z": -1.03125,
+ "stations": "Archer City,Bass Ring,Noli Orbital"
+ },
+ {
+ "name": "LHS 397",
+ "pos_x": 8.5625,
+ "pos_y": 3.875,
+ "pos_z": 16.875,
+ "stations": "Zillig Depot,Larbalestier Beacon,Thornycroft Port"
+ },
+ {
+ "name": "LHS 3976",
+ "pos_x": -90.84375,
+ "pos_y": -52.15625,
+ "pos_z": -24.34375,
+ "stations": "Killough Terminal,Bohnhoff Hub"
+ },
+ {
+ "name": "LHS 3980",
+ "pos_x": -79.09375,
+ "pos_y": -28.125,
+ "pos_z": -26.8125,
+ "stations": "Gresley Enterprise,Parazynski Park,Wilcutt Terminal,Volkov Relay,Schlegel Keep"
+ },
+ {
+ "name": "LHS 4003",
+ "pos_x": -23,
+ "pos_y": -10.9375,
+ "pos_z": -7.4375,
+ "stations": "Womack Holdings,Antonio de Andrade Station"
+ },
+ {
+ "name": "LHS 4019",
+ "pos_x": -56.5625,
+ "pos_y": -36.1875,
+ "pos_z": -17.5625,
+ "stations": "Leibniz Mines,Buffett Works,Robinson Base"
+ },
+ {
+ "name": "LHS 4031",
+ "pos_x": 67.5625,
+ "pos_y": -134.125,
+ "pos_z": 66.84375,
+ "stations": "Garrido Market,Wild Dock,Pryor Base,Gorgani Keep,Baker Landing,Fairey Arena,Hoffmeister Vision,Dixon's Claim"
+ },
+ {
+ "name": "LHS 4032",
+ "pos_x": -19,
+ "pos_y": -59.46875,
+ "pos_z": 6.125,
+ "stations": "Currie's Inheritance,Asher Hub,Russell Landing"
+ },
+ {
+ "name": "LHS 4046",
+ "pos_x": -36.6875,
+ "pos_y": -72.84375,
+ "pos_z": -0.3125,
+ "stations": "Malchiodi Port,Klein Station,Shawl Hub"
+ },
+ {
+ "name": "LHS 4047",
+ "pos_x": -25.40625,
+ "pos_y": -55.09375,
+ "pos_z": 0.0625,
+ "stations": "Walz Terminal,Yurchikhin Enterprise,Miller Installation,Barba Orbital"
+ },
+ {
+ "name": "LHS 4058",
+ "pos_x": 0.375,
+ "pos_y": -40.75,
+ "pos_z": 9.46875,
+ "stations": "Rorschach Hub,Vasquez de Coronado Silo"
+ },
+ {
+ "name": "LHS 411",
+ "pos_x": -13.125,
+ "pos_y": 23.59375,
+ "pos_z": 18.625,
+ "stations": "Shuttleworth Platform,Walker Colony,Al Saud Station,Weyl Beacon"
+ },
+ {
+ "name": "LHS 417",
+ "pos_x": -18.3125,
+ "pos_y": 18.1875,
+ "pos_z": 4.90625,
+ "stations": "Gernhardt Camp,Bohm Palace"
+ },
+ {
+ "name": "LHS 435",
+ "pos_x": -61.96875,
+ "pos_y": 47.46875,
+ "pos_z": 26,
+ "stations": "Wallis Mines"
+ },
+ {
+ "name": "LHS 446",
+ "pos_x": -44.3125,
+ "pos_y": 31.625,
+ "pos_z": 11.15625,
+ "stations": "Ryazanski Terminal"
+ },
+ {
+ "name": "LHS 448",
+ "pos_x": -35.09375,
+ "pos_y": 27.0625,
+ "pos_z": 46.4375,
+ "stations": "Seddon Dock"
+ },
+ {
+ "name": "LHS 449",
+ "pos_x": 4.1875,
+ "pos_y": -1.90625,
+ "pos_z": 14.0625,
+ "stations": "Perry Station,Fisher Point"
+ },
+ {
+ "name": "LHS 450",
+ "pos_x": -12.40625,
+ "pos_y": 7.8125,
+ "pos_z": -1.875,
+ "stations": "Wilson Relay,Darboux Legacy,Stevenson Relay,McKay Prospect"
+ },
+ {
+ "name": "LHS 452",
+ "pos_x": -16.28125,
+ "pos_y": 10.625,
+ "pos_z": 17.78125,
+ "stations": "Davy Gateway,Agassiz Enterprise,Zamyatin's Progress,Behring Gateway,Weber Settlement"
+ },
+ {
+ "name": "LHS 463",
+ "pos_x": -24.96875,
+ "pos_y": 5.75,
+ "pos_z": 45.9375,
+ "stations": "Fadlan Works,Vries Point"
+ },
+ {
+ "name": "LHS 475",
+ "pos_x": 34.375,
+ "pos_y": -24.15625,
+ "pos_z": 30.21875,
+ "stations": "Margulies Installation,Potez Hub,Peral Prospect"
+ },
+ {
+ "name": "LHS 480",
+ "pos_x": 11.21875,
+ "pos_y": -42.40625,
+ "pos_z": 72.71875,
+ "stations": "Stephenson Settlement,Berezovoy Landing,Griggs Plant,Fibonacci's Progress,Deluc Plant"
+ },
+ {
+ "name": "LHS 492",
+ "pos_x": 20.3125,
+ "pos_y": -45.09375,
+ "pos_z": 59.875,
+ "stations": "Lem Port,Payne Installation,Donaldson Orbital"
+ },
+ {
+ "name": "LHS 5072",
+ "pos_x": -33.34375,
+ "pos_y": 15.15625,
+ "pos_z": -27.53125,
+ "stations": "Cook Laboratory,Boas Installation,Lazutkin Hub"
+ },
+ {
+ "name": "LHS 510",
+ "pos_x": -0.8125,
+ "pos_y": -38.40625,
+ "pos_z": 35.96875,
+ "stations": "Grassmann Platform,Liska Enterprise,Stapledon Landing"
+ },
+ {
+ "name": "LHS 5116",
+ "pos_x": 11.15625,
+ "pos_y": 13.59375,
+ "pos_z": -95.3125,
+ "stations": "Britnev Dock"
+ },
+ {
+ "name": "LHS 512",
+ "pos_x": -7.78125,
+ "pos_y": -42.71875,
+ "pos_z": 37.25,
+ "stations": "Halley Prospect,Coulomb Platform"
+ },
+ {
+ "name": "LHS 5163",
+ "pos_x": -28.90625,
+ "pos_y": 88.34375,
+ "pos_z": -71.5,
+ "stations": "Fadlan Base"
+ },
+ {
+ "name": "LHS 5180",
+ "pos_x": 88.53125,
+ "pos_y": 73.6875,
+ "pos_z": -6.9375,
+ "stations": "Ballard Beacon,Mendez Base"
+ },
+ {
+ "name": "LHS 519",
+ "pos_x": 17,
+ "pos_y": -78.0625,
+ "pos_z": 54.46875,
+ "stations": "Clark Dock"
+ },
+ {
+ "name": "LHS 5217a",
+ "pos_x": -2.25,
+ "pos_y": 79.09375,
+ "pos_z": -8.15625,
+ "stations": "Potagos Terminal"
+ },
+ {
+ "name": "LHS 528",
+ "pos_x": -55.6875,
+ "pos_y": -39.875,
+ "pos_z": 4.6875,
+ "stations": "Puleston Prospect,Mendeleev Survey,Cartwright Station"
+ },
+ {
+ "name": "LHS 5287",
+ "pos_x": -36.40625,
+ "pos_y": 48.1875,
+ "pos_z": -0.78125,
+ "stations": "Heng Terminal,McArthur's Reach"
+ },
+ {
+ "name": "LHS 529",
+ "pos_x": -60.375,
+ "pos_y": -30,
+ "pos_z": -4.125,
+ "stations": "Stiegler Dock,Thompson Platform,Cremona Orbital,Holdstock Laboratory"
+ },
+ {
+ "name": "LHS 53",
+ "pos_x": 19.75,
+ "pos_y": 53.21875,
+ "pos_z": 75.0625,
+ "stations": "O'Connor Settlement,Wylie's Claim,Stafford Platform"
+ },
+ {
+ "name": "LHS 5307",
+ "pos_x": -79.59375,
+ "pos_y": 75.53125,
+ "pos_z": -5.5625,
+ "stations": "Mohmand Plant,Heceta Horizons,Shaw Point"
+ },
+ {
+ "name": "LHS 531",
+ "pos_x": 16.03125,
+ "pos_y": -18.0625,
+ "pos_z": 14.375,
+ "stations": "Nelson Terminal"
+ },
+ {
+ "name": "LHS 5333",
+ "pos_x": -14.8125,
+ "pos_y": -3.96875,
+ "pos_z": 102.25,
+ "stations": "Hooke Orbital,Feynman Terminal,Satcher City,Binnie Point,Boe Orbital,Whymper Arsenal,Harris Stop,Bryant Beacon,Lopez de Villalobos Installation,Whitelaw Base,Samuda Landing"
+ },
+ {
+ "name": "LHS 535",
+ "pos_x": -46.625,
+ "pos_y": 6.84375,
+ "pos_z": -20.4375,
+ "stations": "Alexandria Hub"
+ },
+ {
+ "name": "LHS 538",
+ "pos_x": -24.46875,
+ "pos_y": -74.25,
+ "pos_z": 23.1875,
+ "stations": "Jemison Vision,Pytheas Point,Eisele Point"
+ },
+ {
+ "name": "LHS 54",
+ "pos_x": 5.46875,
+ "pos_y": 15.5,
+ "pos_z": 29.75,
+ "stations": "Nelson Dock,Kurland Observatory,Silves Keep,Popper Enterprise"
+ },
+ {
+ "name": "LHS 5404",
+ "pos_x": 17.21875,
+ "pos_y": -108.75,
+ "pos_z": 46.65625,
+ "stations": "Haldeman II Enterprise,Wright Plant,Schommer Port"
+ },
+ {
+ "name": "LHS 547",
+ "pos_x": 0.5,
+ "pos_y": -38.21875,
+ "pos_z": 12.65625,
+ "stations": "Clerk Laboratory,McCoy Landing,Khan Terminal"
+ },
+ {
+ "name": "LHS 55",
+ "pos_x": -23.90625,
+ "pos_y": 39.28125,
+ "pos_z": 34.875,
+ "stations": "Illy Platform,Taylor Keep,Henry Horizons"
+ },
+ {
+ "name": "LHS 6007",
+ "pos_x": -59.09375,
+ "pos_y": -15.5625,
+ "pos_z": -32.625,
+ "stations": "Oppenheimer Terminal,Hopkinson Hub,Peterson Ring,Beckman Port"
+ },
+ {
+ "name": "LHS 6027",
+ "pos_x": -51.96875,
+ "pos_y": -45.4375,
+ "pos_z": -43.46875,
+ "stations": "Joliot-Curie Station,Jemison Port,Powell Base"
+ },
+ {
+ "name": "LHS 6028",
+ "pos_x": -20.46875,
+ "pos_y": -38.46875,
+ "pos_z": -21.75,
+ "stations": "Tryggvason Orbital,Wylie Prospect,Escobar Relay,Braun Point"
+ },
+ {
+ "name": "LHS 6031",
+ "pos_x": 11.3125,
+ "pos_y": 0.15625,
+ "pos_z": 29.03125,
+ "stations": "Farkas Terminal,Volta Hub,Oefelein Terminal,Tudela Point,Fontana Dock,Appel Dock,Polansky Hub,Hopkinson Town,Dobrovolskiy Station"
+ },
+ {
+ "name": "LHS 6050",
+ "pos_x": -13.875,
+ "pos_y": -52.71875,
+ "pos_z": -47.8125,
+ "stations": "Fairbairn Landing,Thoreau City,Pasteur Gateway,Harris Port,Wellman Works,Fleming's Inheritance,Skvortsov Relay,Houtman's Progress,Holland Lab"
+ },
+ {
+ "name": "LHS 6103",
+ "pos_x": 8.84375,
+ "pos_y": 6.03125,
+ "pos_z": -72,
+ "stations": "Weston Orbital,Clayton Point"
+ },
+ {
+ "name": "LHS 6128",
+ "pos_x": -14.4375,
+ "pos_y": 18.3125,
+ "pos_z": -29.28125,
+ "stations": "Olivas Mines,Napier Base,Lyakhov Depot"
+ },
+ {
+ "name": "LHS 6187",
+ "pos_x": 77.6875,
+ "pos_y": 73,
+ "pos_z": -24.1875,
+ "stations": "Molina Horizons,Hooke Gateway,Sabine Barracks"
+ },
+ {
+ "name": "LHS 6197",
+ "pos_x": 79.5,
+ "pos_y": 45,
+ "pos_z": 10.46875,
+ "stations": "Stith Port,Davis Terminal,Clerk Terminal,Roberts Refinery,Nixon's Progress"
+ },
+ {
+ "name": "LHS 6282",
+ "pos_x": -11.46875,
+ "pos_y": 39.78125,
+ "pos_z": 22.78125,
+ "stations": "Dezhurov Terminal,Leeuwenhoek Survey,Heaviside Hub"
+ },
+ {
+ "name": "LHS 6305",
+ "pos_x": -24.53125,
+ "pos_y": 43.28125,
+ "pos_z": 54.25,
+ "stations": "Nielsen Orbital,Schmitz Ring,Finney Observatory,Fernao do Po Dock,Sellings Base"
+ },
+ {
+ "name": "LHS 6309",
+ "pos_x": -33.5625,
+ "pos_y": 33.125,
+ "pos_z": 13.46875,
+ "stations": "Fontana Horizons,Wilson Terminal,Birkhoff Vista,Hutton Station,Tesla Station,Nikolayev Dock,Faraday Station,Hadfield Vision,Cockrell Port,Pippin Landing,Parmitano Horizons,Sellers' Progress"
+ },
+ {
+ "name": "LHS 6326",
+ "pos_x": -32.46875,
+ "pos_y": 15.15625,
+ "pos_z": 91,
+ "stations": "Noether Colony,Semeonis Dock,Shaver Dock"
+ },
+ {
+ "name": "LHS 6386",
+ "pos_x": -54.96875,
+ "pos_y": -5.09375,
+ "pos_z": -3,
+ "stations": "Griffith City,Sekowski Station,Malaspina Port,Tevis Orbital,Kube-McDowell Gateway,Binnie's Progress,Boulle Enterprise,Gregory Port,Viktorenko Hub,Peary Prospect"
+ },
+ {
+ "name": "LHS 64",
+ "pos_x": -76.6875,
+ "pos_y": 11.09375,
+ "pos_z": -10.8125,
+ "stations": "McArthur Colony,Wiberg Hangar,Doyle Silo"
+ },
+ {
+ "name": "LHS 6408",
+ "pos_x": -62.84375,
+ "pos_y": -13.90625,
+ "pos_z": -10.8125,
+ "stations": "Antonelli Platform,Goeppert-Mayer Port,Roosa Terminal,Yurchikhin Depot"
+ },
+ {
+ "name": "LHS 6410",
+ "pos_x": -43.96875,
+ "pos_y": -27.3125,
+ "pos_z": 1.8125,
+ "stations": "Doyle Base,Lockhart Dock,Nelson Landing"
+ },
+ {
+ "name": "LHS 6427",
+ "pos_x": -33.25,
+ "pos_y": -19.78125,
+ "pos_z": -9.53125,
+ "stations": "Knight Orbital,Marconi Works,Bohr Dock,Kanwar Station"
+ },
+ {
+ "name": "LHS 71",
+ "pos_x": -20.03125,
+ "pos_y": -1.15625,
+ "pos_z": -7.40625,
+ "stations": "Jun Hub,Goeschke Dock,Tayler Bastion,Frick Port"
+ },
+ {
+ "name": "Li Bende",
+ "pos_x": 105.3125,
+ "pos_y": -44.15625,
+ "pos_z": 26.71875,
+ "stations": "Naburimannu Enterprise,Werber Penal colony,Ziegel Ring,Walker Camp"
+ },
+ {
+ "name": "Li Bestina",
+ "pos_x": 20.875,
+ "pos_y": -13.34375,
+ "pos_z": 92.4375,
+ "stations": "Kress Enterprise,Garcia Beacon,Bacigalupi Orbital,Stanley Enterprise,Neumann Refinery"
+ },
+ {
+ "name": "Li Buj",
+ "pos_x": -23.8125,
+ "pos_y": 72.6875,
+ "pos_z": 122.46875,
+ "stations": "Teng's Claim,Dantec Mine"
+ },
+ {
+ "name": "Li Cha",
+ "pos_x": 15.125,
+ "pos_y": -44.5625,
+ "pos_z": -63.40625,
+ "stations": "Bosch City,Moore Survey,Archer Enterprise"
+ },
+ {
+ "name": "Li Chen Yun",
+ "pos_x": -12.65625,
+ "pos_y": 88.625,
+ "pos_z": -154.21875,
+ "stations": "Baudin Relay,Rochon Horizons"
+ },
+ {
+ "name": "Li Cherbon",
+ "pos_x": -182.65625,
+ "pos_y": 21.125,
+ "pos_z": -16,
+ "stations": "Martins Landing,Ledyard Arsenal"
+ },
+ {
+ "name": "Li Chi",
+ "pos_x": 129.78125,
+ "pos_y": -164.0625,
+ "pos_z": 53.5625,
+ "stations": "Gora Hub"
+ },
+ {
+ "name": "Li Chiutama",
+ "pos_x": 83.9375,
+ "pos_y": -26.125,
+ "pos_z": 150.40625,
+ "stations": "Henslow Legacy,Veblen Relay"
+ },
+ {
+ "name": "Li Chong",
+ "pos_x": 60.3125,
+ "pos_y": -63.34375,
+ "pos_z": 26.125,
+ "stations": "Jernigan Platform,Ostrander Works"
+ },
+ {
+ "name": "Li Chotep",
+ "pos_x": 156.96875,
+ "pos_y": -2.71875,
+ "pos_z": 8.46875,
+ "stations": "Smeaton Settlement,Behring Orbital,Bruce Beacon,Stokes Installation"
+ },
+ {
+ "name": "Li Chu Pard",
+ "pos_x": 20.65625,
+ "pos_y": -97.5625,
+ "pos_z": 171.3125,
+ "stations": "Eddington Dock,Pajdusakova Orbital"
+ },
+ {
+ "name": "Li Chul",
+ "pos_x": 120.03125,
+ "pos_y": 60.21875,
+ "pos_z": 44.71875,
+ "stations": "Dobrovolskiy Ring,Scobee Station,Wakata Port,Chretien Terminal,Foale Orbital,Cooper Base,Nansen Enterprise,Cardano Camp"
+ },
+ {
+ "name": "Li Hai",
+ "pos_x": -108.8125,
+ "pos_y": -84.9375,
+ "pos_z": -98.75,
+ "stations": "Plucker Mines,Francisco de Eliza Settlement,Franklin Mines,Dupuy de Lome Settlement"
+ },
+ {
+ "name": "Li Hsi Suix",
+ "pos_x": 192.40625,
+ "pos_y": -117.875,
+ "pos_z": 74.03125,
+ "stations": "Gentil Outpost"
+ },
+ {
+ "name": "Li Jiu",
+ "pos_x": 23.65625,
+ "pos_y": -238.125,
+ "pos_z": -12.25,
+ "stations": "Naubakht Dock,Foerster Port,Lunney Station,Bertin Observatory"
+ },
+ {
+ "name": "Li Jungu",
+ "pos_x": -0.09375,
+ "pos_y": -59.25,
+ "pos_z": 120.5,
+ "stations": "Musgrave Prospect,Dantec Terminal,Ziemianski Hub,Petaja Enterprise,Atiyah Depot"
+ },
+ {
+ "name": "Li K'anja",
+ "pos_x": 105.1875,
+ "pos_y": 125.15625,
+ "pos_z": 38.0625,
+ "stations": "Lee Port,Napier Relay"
+ },
+ {
+ "name": "Li Na",
+ "pos_x": -23.625,
+ "pos_y": -98.5,
+ "pos_z": -76.625,
+ "stations": "Friend Settlement,Caidin Point,Mitchell Relay"
+ },
+ {
+ "name": "Li Nang Yi",
+ "pos_x": 96.34375,
+ "pos_y": -31.875,
+ "pos_z": 117.9375,
+ "stations": "Grant Dock,Velho City,Barentsz Gateway,Stasheff Landing"
+ },
+ {
+ "name": "Li Neb Tun",
+ "pos_x": -24.625,
+ "pos_y": 21.3125,
+ "pos_z": -141.5,
+ "stations": "Moisuc Mines,Hughes-Fulford Works"
+ },
+ {
+ "name": "Li Nones",
+ "pos_x": -19.21875,
+ "pos_y": -88.53125,
+ "pos_z": 134.75,
+ "stations": "Kotelnikov City"
+ },
+ {
+ "name": "Li Nongal",
+ "pos_x": -7.78125,
+ "pos_y": 27.15625,
+ "pos_z": 141.625,
+ "stations": "Hoyle Settlement,Kuttner Mines"
+ },
+ {
+ "name": "Li Pa Pai",
+ "pos_x": 124.6875,
+ "pos_y": 60.8125,
+ "pos_z": 40.03125,
+ "stations": "Bunch Asylum"
+ },
+ {
+ "name": "Li Prope",
+ "pos_x": 91.28125,
+ "pos_y": 62.53125,
+ "pos_z": -111.0625,
+ "stations": "Goeschke Station"
+ },
+ {
+ "name": "Li Quang",
+ "pos_x": -108.03125,
+ "pos_y": 36.84375,
+ "pos_z": -11.75,
+ "stations": "Al-Battani Relay"
+ },
+ {
+ "name": "Li Shipek",
+ "pos_x": 108.1875,
+ "pos_y": -153.125,
+ "pos_z": 162.40625,
+ "stations": "Mohr Port,Ferguson Outpost"
+ },
+ {
+ "name": "Li Szu",
+ "pos_x": 29.59375,
+ "pos_y": -91.65625,
+ "pos_z": 52.5,
+ "stations": "Meuron Station,Curtiss Ring,Holub Enterprise"
+ },
+ {
+ "name": "Li Tan",
+ "pos_x": 92.09375,
+ "pos_y": -30.875,
+ "pos_z": 54.9375,
+ "stations": "Good City"
+ },
+ {
+ "name": "Li Tenyayp",
+ "pos_x": -126.4375,
+ "pos_y": 7.84375,
+ "pos_z": -66,
+ "stations": "Lysenko Port,Citroen Mine,Weber Beacon"
+ },
+ {
+ "name": "Li Trytho",
+ "pos_x": 140.96875,
+ "pos_y": -79.40625,
+ "pos_z": -41.78125,
+ "stations": "Abel Works"
+ },
+ {
+ "name": "Li Tzicnii",
+ "pos_x": -54.71875,
+ "pos_y": -23.15625,
+ "pos_z": -56,
+ "stations": "Teller Ring,Ramelli Terminal,Singer Colony"
+ },
+ {
+ "name": "Liabeze",
+ "pos_x": 72.46875,
+ "pos_y": -121.5,
+ "pos_z": 26.21875,
+ "stations": "Cheranovsky Dock,Lyulka Beacon,Mandel Penal colony"
+ },
+ {
+ "name": "Liaedin",
+ "pos_x": -32.03125,
+ "pos_y": -5.8125,
+ "pos_z": 38.125,
+ "stations": "Kohl Terminal,Peters Station,Fortress Anderton,Tavares Port,Kuiper Vision,Moore Exchange"
+ },
+ {
+ "name": "Libaia Bara",
+ "pos_x": -56.9375,
+ "pos_y": 80.9375,
+ "pos_z": -3.15625,
+ "stations": "Peary Refinery,Le Guin Plant,Clapperton Dock,Perga Port"
+ },
+ {
+ "name": "Libanatya",
+ "pos_x": 109.625,
+ "pos_y": -170.5,
+ "pos_z": 69.25,
+ "stations": "Potez Platform,Wang Stop"
+ },
+ {
+ "name": "Libarai",
+ "pos_x": -4.8125,
+ "pos_y": 103.4375,
+ "pos_z": -134.34375,
+ "stations": "von Bellingshausen Outpost,Bear Outpost,Vlamingh Settlement"
+ },
+ {
+ "name": "Libuhchal",
+ "pos_x": 78.4375,
+ "pos_y": -229.59375,
+ "pos_z": 11.46875,
+ "stations": "Balog's Claim,Anthony de la Roche Hub"
+ },
+ {
+ "name": "Libumij",
+ "pos_x": 170.75,
+ "pos_y": 44.125,
+ "pos_z": -13.59375,
+ "stations": "Preuss Vision,Morris Horizons,Dedman Vision,Jacobi Keep"
+ },
+ {
+ "name": "Liburodo",
+ "pos_x": 23.75,
+ "pos_y": -12.4375,
+ "pos_z": 56.03125,
+ "stations": "Landsteiner Mines,Thurston Depot"
+ },
+ {
+ "name": "Liburumni",
+ "pos_x": 138.8125,
+ "pos_y": -33.53125,
+ "pos_z": 78.9375,
+ "stations": "Caidin Ring,Wilson Orbital,Alpers Gateway"
+ },
+ {
+ "name": "Lida",
+ "pos_x": -96.03125,
+ "pos_y": -23.28125,
+ "pos_z": 118.90625,
+ "stations": "Boyle Platform,Murray Settlement,Herrington Hub,Olsen's Progress"
+ },
+ {
+ "name": "Lidals",
+ "pos_x": 78.8125,
+ "pos_y": -81.1875,
+ "pos_z": -20.25,
+ "stations": "Adragna Mines,Wilhelm von Struve Mines,Silves Holdings"
+ },
+ {
+ "name": "Lidar",
+ "pos_x": 116.84375,
+ "pos_y": -69.625,
+ "pos_z": 114.71875,
+ "stations": "Regiomontanus Holdings,Aikin Hub,Hind City"
+ },
+ {
+ "name": "Lidpar",
+ "pos_x": -64.0625,
+ "pos_y": -53.96875,
+ "pos_z": -13.8125,
+ "stations": "Carlisle Station,Divis Terminal"
+ },
+ {
+ "name": "Lie Yu Kou",
+ "pos_x": -63.34375,
+ "pos_y": -27.15625,
+ "pos_z": -66,
+ "stations": "Baird Mine"
+ },
+ {
+ "name": "Lie Yupik",
+ "pos_x": 98.8125,
+ "pos_y": 15.375,
+ "pos_z": -35.1875,
+ "stations": "Bachman Dock"
+ },
+ {
+ "name": "Lie Zha",
+ "pos_x": -82.875,
+ "pos_y": 117.75,
+ "pos_z": -84.71875,
+ "stations": "Barnes Mine"
+ },
+ {
+ "name": "Lie Zhangwe",
+ "pos_x": 63.5,
+ "pos_y": -152.96875,
+ "pos_z": -62.84375,
+ "stations": "Babakin Legacy"
+ },
+ {
+ "name": "Lie Zhi",
+ "pos_x": 137.59375,
+ "pos_y": -15,
+ "pos_z": -46.53125,
+ "stations": "King Terminal"
+ },
+ {
+ "name": "Lie Zhonpon",
+ "pos_x": 79.96875,
+ "pos_y": 174.28125,
+ "pos_z": 42.34375,
+ "stations": "al-Khayyam's Claim"
+ },
+ {
+ "name": "Lie Zip",
+ "pos_x": 16.5,
+ "pos_y": 40.375,
+ "pos_z": -67.3125,
+ "stations": "Nearchus Installation,Stabenow Settlement,Mitchell Colony"
+ },
+ {
+ "name": "Lieh Tzu",
+ "pos_x": -95.75,
+ "pos_y": -66.65625,
+ "pos_z": 130.625,
+ "stations": "Rocklynne Port,Kress Hub"
+ },
+ {
+ "name": "Lieldi",
+ "pos_x": 167.9375,
+ "pos_y": -117.34375,
+ "pos_z": 33.8125,
+ "stations": "Filippenko Platform,Akers Vision,Sheremetevsky Prospect"
+ },
+ {
+ "name": "Lieledos",
+ "pos_x": -89.375,
+ "pos_y": -11.40625,
+ "pos_z": 70.21875,
+ "stations": "Metz Mine,Froude Mines"
+ },
+ {
+ "name": "Lien",
+ "pos_x": 94.9375,
+ "pos_y": 81.0625,
+ "pos_z": 85.78125,
+ "stations": "Wang Depot"
+ },
+ {
+ "name": "Lienate",
+ "pos_x": 165.96875,
+ "pos_y": 34.71875,
+ "pos_z": 59.40625,
+ "stations": "Macgregor Colony,Samuda Refinery"
+ },
+ {
+ "name": "Lifthruti",
+ "pos_x": 70.15625,
+ "pos_y": -143.625,
+ "pos_z": 123.15625,
+ "stations": "Lewis' Exile"
+ },
+ {
+ "name": "Likedeeler Magna Libertas",
+ "pos_x": 27.25,
+ "pos_y": -184.875,
+ "pos_z": 48.71875,
+ "stations": "Kaluta Station,Reinmuth Dock,Hampson Station,Jekhowsky Ring,Perlmutter Market,Ocampo Enterprise,Johri City,Glushko City,Goulart Silo,Dayuan Horizons"
+ },
+ {
+ "name": "Likohochna",
+ "pos_x": 113.21875,
+ "pos_y": 21.59375,
+ "pos_z": -55.96875,
+ "stations": "Leestma Settlement,Aksyonov Colony,Readdy Settlement,Walter Platform,Mallory Installation"
+ },
+ {
+ "name": "Likokutha",
+ "pos_x": 115.15625,
+ "pos_y": -157.5,
+ "pos_z": -21.5,
+ "stations": "Stephan Prospect,Maury Hub"
+ },
+ {
+ "name": "Lileni",
+ "pos_x": -11.34375,
+ "pos_y": -136,
+ "pos_z": -13.75,
+ "stations": "Wachmann Vision,Maanen Gateway,Key Installation,Roelofs Terminal"
+ },
+ {
+ "name": "Lillith",
+ "pos_x": -11.375,
+ "pos_y": 25.28125,
+ "pos_z": 84.875,
+ "stations": "Alas Installation"
+ },
+ {
+ "name": "Limani",
+ "pos_x": 100.9375,
+ "pos_y": -3.34375,
+ "pos_z": 1.875,
+ "stations": "Satcher Vision"
+ },
+ {
+ "name": "Limapa",
+ "pos_x": -46.3125,
+ "pos_y": -44.03125,
+ "pos_z": 105.53125,
+ "stations": "Kovalyonok Port,Feustel Landing,Arber Hub"
+ },
+ {
+ "name": "Limi",
+ "pos_x": -118.46875,
+ "pos_y": 3.40625,
+ "pos_z": 53.875,
+ "stations": "Perez Reach,Markham Survey,Park's Folly"
+ },
+ {
+ "name": "Lindol",
+ "pos_x": -17.6875,
+ "pos_y": 86.6875,
+ "pos_z": 2.8125,
+ "stations": "Stein Terminal"
+ },
+ {
+ "name": "Ling Deti",
+ "pos_x": 79.28125,
+ "pos_y": -93.59375,
+ "pos_z": 4.53125,
+ "stations": "Youll Vision,Young Station,Farmer Laboratory"
+ },
+ {
+ "name": "Linghus",
+ "pos_x": -46.34375,
+ "pos_y": -70.0625,
+ "pos_z": -12.625,
+ "stations": "Danjon Arsenal,Clapperton Dock,Elwood's Folly"
+ },
+ {
+ "name": "Linksmine",
+ "pos_x": 54.28125,
+ "pos_y": 86.5625,
+ "pos_z": -34.71875,
+ "stations": "McCormick City,Siodmak Installation,Korzun Hub"
+ },
+ {
+ "name": "Lisaaka",
+ "pos_x": 202.5625,
+ "pos_y": -173.96875,
+ "pos_z": 12.15625,
+ "stations": "Grunsfeld Beacon,Margulies Arsenal,McDermott Vision"
+ },
+ {
+ "name": "Lisang",
+ "pos_x": 48.53125,
+ "pos_y": -10.0625,
+ "pos_z": 86.9375,
+ "stations": "Fadlan Dock,McCaffrey Market,Bryusov Gateway,Abel Orbital,Vance Terminal"
+ },
+ {
+ "name": "Lisun",
+ "pos_x": 107.875,
+ "pos_y": -33.28125,
+ "pos_z": -63.03125,
+ "stations": "Bradshaw Landing,Ross Colony,Payne Port"
+ },
+ {
+ "name": "Liu Anai",
+ "pos_x": 135.125,
+ "pos_y": -184.09375,
+ "pos_z": -59.21875,
+ "stations": "Hay's Claim"
+ },
+ {
+ "name": "Liu Anan",
+ "pos_x": -48.53125,
+ "pos_y": -126.5625,
+ "pos_z": 74.3125,
+ "stations": "Bohr Port,Zhukovsky Gateway,Michell Vision"
+ },
+ {
+ "name": "Liu Baja",
+ "pos_x": -52.6875,
+ "pos_y": 0.46875,
+ "pos_z": 36.6875,
+ "stations": "Ray Dock,Anderson Depot,Henry Orbital,Reilly Settlement,Bluford Vision"
+ },
+ {
+ "name": "Liu Bajara",
+ "pos_x": 89.40625,
+ "pos_y": 6.3125,
+ "pos_z": -20.0625,
+ "stations": "Tepper Base,Silva Terminal,Anders Penal colony,Bachman Orbital,de Sousa Dock"
+ },
+ {
+ "name": "Liu Baji",
+ "pos_x": -109.40625,
+ "pos_y": 13.96875,
+ "pos_z": 22.59375,
+ "stations": "Williams Survey"
+ },
+ {
+ "name": "Liu Behueno",
+ "pos_x": 76.5625,
+ "pos_y": -124.15625,
+ "pos_z": -81.53125,
+ "stations": "Hughes Arena"
+ },
+ {
+ "name": "Liu Beni",
+ "pos_x": -96.09375,
+ "pos_y": -11.21875,
+ "pos_z": -135.25,
+ "stations": "Davis Settlement,McCoy Survey,Hooke's Progress"
+ },
+ {
+ "name": "Liu Bese",
+ "pos_x": 123.6875,
+ "pos_y": -2.3125,
+ "pos_z": -134.5625,
+ "stations": "Narbeth Relay,Robinson Camp"
+ },
+ {
+ "name": "Liu Beserka",
+ "pos_x": 152.1875,
+ "pos_y": -105.53125,
+ "pos_z": 37.3125,
+ "stations": "Dreyer's Inheritance"
+ },
+ {
+ "name": "Liu Di",
+ "pos_x": 61.40625,
+ "pos_y": -141.21875,
+ "pos_z": 15.96875,
+ "stations": "Sudworth Orbital,Wenzel Survey,Dittmar Port,Espenak Ring,Ehrenfried Kegel Relay"
+ },
+ {
+ "name": "Liu Hef",
+ "pos_x": -101.59375,
+ "pos_y": 56.09375,
+ "pos_z": -59.90625,
+ "stations": "Davis Horizons"
+ },
+ {
+ "name": "Liu Hesan",
+ "pos_x": 71.5,
+ "pos_y": -143.21875,
+ "pos_z": 145.28125,
+ "stations": "Clark Hub,Tedin Port,Hennepin Vision"
+ },
+ {
+ "name": "Liu Huang",
+ "pos_x": -87.4375,
+ "pos_y": 54.28125,
+ "pos_z": -73.4375,
+ "stations": "Clerk Vision,McDivitt Dock"
+ },
+ {
+ "name": "Liu Hut",
+ "pos_x": 170.71875,
+ "pos_y": -52.03125,
+ "pos_z": 47.71875,
+ "stations": "Bracewell Station,Hyakutake Port"
+ },
+ {
+ "name": "Liu Links",
+ "pos_x": 78.03125,
+ "pos_y": -2.5,
+ "pos_z": 49.125,
+ "stations": "Stevin Dock,Grothendieck Depot,Paczynski Prospect,Beaufoy Gateway"
+ },
+ {
+ "name": "Liu Pei",
+ "pos_x": 57,
+ "pos_y": -40.625,
+ "pos_z": -103.84375,
+ "stations": "Smith Outpost"
+ },
+ {
+ "name": "Liu Xians",
+ "pos_x": 107.21875,
+ "pos_y": 13.5,
+ "pos_z": -79.25,
+ "stations": "Scobee Terminal,Michelson Colony"
+ },
+ {
+ "name": "Liu Xingga",
+ "pos_x": 11.3125,
+ "pos_y": -76.53125,
+ "pos_z": -79.96875,
+ "stations": "Pournelle Enterprise,Holland Enterprise,Ashton Station,Scalzi Port,Jenkinson Dock,Great Port,Sharp Port,MacLean Hub"
+ },
+ {
+ "name": "Liu Xuan De",
+ "pos_x": 173.90625,
+ "pos_y": -188.1875,
+ "pos_z": 39.03125,
+ "stations": "Zeppelin Landing"
+ },
+ {
+ "name": "Liu Yamici",
+ "pos_x": -15.96875,
+ "pos_y": -176.75,
+ "pos_z": 32,
+ "stations": "Dolgov Vision,Vasilyev Arsenal,Yamamoto Terminal,Riccioli Port"
+ },
+ {
+ "name": "Liu Yanec",
+ "pos_x": -82.1875,
+ "pos_y": -2.125,
+ "pos_z": -77.09375,
+ "stations": "Haber Dock,Kurland Dock,Vela Port"
+ },
+ {
+ "name": "Liu Yines",
+ "pos_x": 124.25,
+ "pos_y": 19.21875,
+ "pos_z": -55.40625,
+ "stations": "Baraniecki Orbital,Lagrange Enterprise,Champlain Installation,Russell Laboratory"
+ },
+ {
+ "name": "Liv",
+ "pos_x": 9.53125,
+ "pos_y": -169.34375,
+ "pos_z": 103.40625,
+ "stations": "Furukawa Platform,Edmondson Station,Goldschmidt Prospect,Janifer Beacon"
+ },
+ {
+ "name": "Llwydanane",
+ "pos_x": 23.96875,
+ "pos_y": -22.6875,
+ "pos_z": 149.375,
+ "stations": "Leclerc Vision,Carey Landing,Kratman Penal colony"
+ },
+ {
+ "name": "Llwyddi Di",
+ "pos_x": -36,
+ "pos_y": 0.90625,
+ "pos_z": -8.03125,
+ "stations": "Vetulani Platform,Behring Base"
+ },
+ {
+ "name": "Llyr",
+ "pos_x": 50.125,
+ "pos_y": -40.96875,
+ "pos_z": 43.0625,
+ "stations": "Baraniecki Vision,Cabral Camp,Mattei Landing"
+ },
+ {
+ "name": "LO Muscae",
+ "pos_x": 98.96875,
+ "pos_y": -7.5,
+ "pos_z": 63.84375,
+ "stations": "Crown's Inheritance,Carey Settlement,Avdeyev City,Butz Hub,Bates Holdings"
+ },
+ {
+ "name": "LO Pegasi",
+ "pos_x": -73.25,
+ "pos_y": -28.09375,
+ "pos_z": 19.75,
+ "stations": "Garcia Station,Grijalva Survey,Dashiell Terminal"
+ },
+ {
+ "name": "Lobed",
+ "pos_x": 74.5625,
+ "pos_y": -103.25,
+ "pos_z": -21.90625,
+ "stations": "Scortia Depot"
+ },
+ {
+ "name": "Lobeduning",
+ "pos_x": -120.5,
+ "pos_y": -108.71875,
+ "pos_z": -29.4375,
+ "stations": "Harris Orbital,Plucker Platform,Drummond Port,Cooper Depot,Krylov Vision"
+ },
+ {
+ "name": "Lobetati",
+ "pos_x": 83.375,
+ "pos_y": -242.71875,
+ "pos_z": 58.75,
+ "stations": "Rabinowitz Asylum"
+ },
+ {
+ "name": "Lobices",
+ "pos_x": -109.53125,
+ "pos_y": -27.8125,
+ "pos_z": 111.0625,
+ "stations": "Schmitz Landing"
+ },
+ {
+ "name": "Lodemovoi",
+ "pos_x": -67.4375,
+ "pos_y": -12.25,
+ "pos_z": -27.21875,
+ "stations": "Lysenko Platform,Dobrovolski Prospect"
+ },
+ {
+ "name": "Lodemowo",
+ "pos_x": 144.65625,
+ "pos_y": -63.21875,
+ "pos_z": -77.4375,
+ "stations": "Bluford Hub,Love City,Allen Settlement,Neff Depot"
+ },
+ {
+ "name": "Lodere",
+ "pos_x": 3,
+ "pos_y": -0.5,
+ "pos_z": -135.8125,
+ "stations": "Siemens Horizons"
+ },
+ {
+ "name": "Loderebinar",
+ "pos_x": -64.59375,
+ "pos_y": 138.84375,
+ "pos_z": 40.90625,
+ "stations": "Charnas Settlement,Waldrop Platform,Kirtley's Folly"
+ },
+ {
+ "name": "Lodes",
+ "pos_x": 115.71875,
+ "pos_y": -169.28125,
+ "pos_z": 136.15625,
+ "stations": "Giancola Depot,Cady Laboratory"
+ },
+ {
+ "name": "Lodhary",
+ "pos_x": -107.75,
+ "pos_y": -96.96875,
+ "pos_z": 20.875,
+ "stations": "Lagrange Gateway,Coppel Enterprise,Anthony Dock,Carter Refinery"
+ },
+ {
+ "name": "Lodheima",
+ "pos_x": -11.34375,
+ "pos_y": -24.46875,
+ "pos_z": -122.75,
+ "stations": "Ocampo Landing"
+ },
+ {
+ "name": "Loga",
+ "pos_x": -79.78125,
+ "pos_y": 36.53125,
+ "pos_z": -42.0625,
+ "stations": "Keyes Platform,Bertin Plant,Carlisle Settlement,Asprin Beacon"
+ },
+ {
+ "name": "Logon",
+ "pos_x": 120.71875,
+ "pos_y": 97.09375,
+ "pos_z": -16.34375,
+ "stations": "Bombelli Horizons,Garfinkle Installation,Kelly City"
+ },
+ {
+ "name": "Logoni",
+ "pos_x": 69.21875,
+ "pos_y": 29.03125,
+ "pos_z": 17.28125,
+ "stations": "Burnell Vision"
+ },
+ {
+ "name": "Loha",
+ "pos_x": 78.78125,
+ "pos_y": 10.90625,
+ "pos_z": -49.46875,
+ "stations": "Whitworth Station,Lanier Base,Elion Orbital,Kovalevskaya Silo"
+ },
+ {
+ "name": "Lohan",
+ "pos_x": 133.21875,
+ "pos_y": -62.1875,
+ "pos_z": 57.5,
+ "stations": "Connes Port,Carr Depot"
+ },
+ {
+ "name": "Loharii",
+ "pos_x": -64.84375,
+ "pos_y": 46.6875,
+ "pos_z": -78.6875,
+ "stations": "Dorsett Port,Dobzhansky Port,Gooch Penal colony,Spedding Terminal"
+ },
+ {
+ "name": "Lohra",
+ "pos_x": 19.0625,
+ "pos_y": -134.5625,
+ "pos_z": -54.03125,
+ "stations": "Schomburg Colony"
+ },
+ {
+ "name": "Lokaangina",
+ "pos_x": 27.71875,
+ "pos_y": -177.4375,
+ "pos_z": 134.1875,
+ "stations": "Kirby Terminal,Woodroffe Station,Pike Oasis"
+ },
+ {
+ "name": "Lokaantii",
+ "pos_x": 129.625,
+ "pos_y": -4.1875,
+ "pos_z": 11.875,
+ "stations": "Hawking Landing,Tepper Freeport,Derleth Landing,Shalatula Plant"
+ },
+ {
+ "name": "Lokans",
+ "pos_x": 75.1875,
+ "pos_y": 50.28125,
+ "pos_z": -70.65625,
+ "stations": "Searfoss Relay,Hurley Camp"
+ },
+ {
+ "name": "Lokapuri",
+ "pos_x": -72.78125,
+ "pos_y": 33.78125,
+ "pos_z": 1.9375,
+ "stations": "Yegorov Terminal,Phillips Terminal,Bernoulli Port"
+ },
+ {
+ "name": "Loki Tan",
+ "pos_x": 32.375,
+ "pos_y": 28.34375,
+ "pos_z": -109.375,
+ "stations": "Worden City,Irvin City,Harrison Forum,Wescott Plant,Thirsk Ring"
+ },
+ {
+ "name": "Loki Tstae",
+ "pos_x": -83.28125,
+ "pos_y": -22.0625,
+ "pos_z": 138.4375,
+ "stations": "McCandless Terminal"
+ },
+ {
+ "name": "Lokipi",
+ "pos_x": -2.09375,
+ "pos_y": -68.5,
+ "pos_z": -90.84375,
+ "stations": "Reed Installation,Laird Penal colony,Pond Platform"
+ },
+ {
+ "name": "Lokit",
+ "pos_x": 96.21875,
+ "pos_y": 0.21875,
+ "pos_z": 26.4375,
+ "stations": "Henricks Settlement"
+ },
+ {
+ "name": "Lokitaka Mu",
+ "pos_x": -19.09375,
+ "pos_y": -11.75,
+ "pos_z": 51.40625,
+ "stations": "MacLean Dock,Forstchen Landing,Turzillo Plant"
+ },
+ {
+ "name": "Lokites",
+ "pos_x": -113.78125,
+ "pos_y": -8.90625,
+ "pos_z": 41.90625,
+ "stations": "Good Observatory,Rawat Retreat"
+ },
+ {
+ "name": "Loko O",
+ "pos_x": 67.0625,
+ "pos_y": 56.75,
+ "pos_z": 117.96875,
+ "stations": "Savitskaya Hub,Illy Dock,Hume Stop"
+ },
+ {
+ "name": "Loko Oh",
+ "pos_x": 104.8125,
+ "pos_y": -160.59375,
+ "pos_z": -14.96875,
+ "stations": "Lagrange Hub,Al Sufi Port,South Port,Eckford Barracks,Nomen Installation"
+ },
+ {
+ "name": "Lokoko",
+ "pos_x": -122.75,
+ "pos_y": -37.0625,
+ "pos_z": -62.96875,
+ "stations": "d'Eyncourt Prospect"
+ },
+ {
+ "name": "Lomba",
+ "pos_x": -59.1875,
+ "pos_y": 26.84375,
+ "pos_z": 82.28125,
+ "stations": "Noriega Hangar,Lobachevsky Survey,Halley Settlement"
+ },
+ {
+ "name": "Lombi",
+ "pos_x": 59.9375,
+ "pos_y": -132.53125,
+ "pos_z": 1.78125,
+ "stations": "Xin Hub,Lubbock Dock,Jenkins Relay,Young Hub"
+ },
+ {
+ "name": "Lombiku",
+ "pos_x": -135.1875,
+ "pos_y": 11.28125,
+ "pos_z": 100.53125,
+ "stations": "Buckey Terminal,Springer Landing,Vlamingh Settlement"
+ },
+ {
+ "name": "Lombor",
+ "pos_x": -55.0625,
+ "pos_y": -69.53125,
+ "pos_z": -14,
+ "stations": "Gaspar de Portola Terminal,Clifton Landing,Blish Depot,Gromov Orbital,Wyndham Terminal,Poincare Terminal,Lobachevsky Dock,Utley Hub,Viete Penal colony,Bachman Prospect"
+ },
+ {
+ "name": "Lomote",
+ "pos_x": 164.40625,
+ "pos_y": -76.09375,
+ "pos_z": -7.125,
+ "stations": "Kawasato Landing,Pippin Settlement,Parkinson Landing"
+ },
+ {
+ "name": "Lomoth",
+ "pos_x": 102.78125,
+ "pos_y": -88.5625,
+ "pos_z": -80.75,
+ "stations": "Weizsacker Retreat"
+ },
+ {
+ "name": "Lomotwa",
+ "pos_x": 60.5625,
+ "pos_y": 7.625,
+ "pos_z": 144.5625,
+ "stations": "Sadi Carnot Point,Klimuk Outpost"
+ },
+ {
+ "name": "Long",
+ "pos_x": 31.6875,
+ "pos_y": -89.5,
+ "pos_z": 17.84375,
+ "stations": "Grushin Prospect"
+ },
+ {
+ "name": "Long Mu",
+ "pos_x": 43.5625,
+ "pos_y": 93.5,
+ "pos_z": 74.25,
+ "stations": "Payton Port,Meikle Holdings,Mendel Terminal,Herschel Relay"
+ },
+ {
+ "name": "Longhus",
+ "pos_x": 63.15625,
+ "pos_y": -94.75,
+ "pos_z": -75.9375,
+ "stations": "Lintott Depot,Dubyago Port,Karachkina Prospect"
+ },
+ {
+ "name": "Lopemakinn",
+ "pos_x": 100.90625,
+ "pos_y": -8.21875,
+ "pos_z": -118.46875,
+ "stations": "Sinclair Port,Qurra's Claim,Cavendish Camp"
+ },
+ {
+ "name": "Lopemba",
+ "pos_x": 34.71875,
+ "pos_y": -122.4375,
+ "pos_z": -43.5,
+ "stations": "Silva Base,Burgess Platform,Cerulli Vision,Baird Platform"
+ },
+ {
+ "name": "Loperada",
+ "pos_x": -102.78125,
+ "pos_y": 97.40625,
+ "pos_z": -4.0625,
+ "stations": "Bloch Vision,Herreshoff Terminal,Fremont Installation,Young Ring,McQuay Relay"
+ },
+ {
+ "name": "Lopo Vuh",
+ "pos_x": 100.40625,
+ "pos_y": -224.625,
+ "pos_z": 32.25,
+ "stations": "Hill Tinsley Station"
+ },
+ {
+ "name": "Lopocareni",
+ "pos_x": -96.71875,
+ "pos_y": -67,
+ "pos_z": 115.5625,
+ "stations": "Watts Stop"
+ },
+ {
+ "name": "Lopocares",
+ "pos_x": 110.59375,
+ "pos_y": 23.90625,
+ "pos_z": 1.75,
+ "stations": "Malenchenko Gateway,Babbage Orbital,Hilbert Enterprise,O'Connor Station,Yegorov Dock,Peterson Gateway,Ockels Enterprise,Auer's Folly,Feynman Terminal,Beltrami Lab,Ahern Hub,Yourkevitch Installation"
+ },
+ {
+ "name": "Loponnaruai",
+ "pos_x": -100.90625,
+ "pos_y": -99.125,
+ "pos_z": -81.84375,
+ "stations": "Crouch Beacon"
+ },
+ {
+ "name": "Loptanii",
+ "pos_x": 7.59375,
+ "pos_y": -259.84375,
+ "pos_z": 80.25,
+ "stations": "Islam Platform,Vyssotsky Outpost,Wolszczan Base"
+ },
+ {
+ "name": "Loptaroju",
+ "pos_x": -68.125,
+ "pos_y": -179.9375,
+ "pos_z": 41.09375,
+ "stations": "RenenBellot Bastion,Dampier Platform,Frimout Landing,Nicholson Port"
+ },
+ {
+ "name": "Lopterones",
+ "pos_x": 182.71875,
+ "pos_y": -140.875,
+ "pos_z": 4,
+ "stations": "Kondo Hub,Adams Landing,Ashbrook Dock"
+ },
+ {
+ "name": "Lopu Manu",
+ "pos_x": -84.84375,
+ "pos_y": 119.78125,
+ "pos_z": -27.03125,
+ "stations": "von Krusenstern Landing"
+ },
+ {
+ "name": "Lopu Maris",
+ "pos_x": 23.96875,
+ "pos_y": -15,
+ "pos_z": 15.21875,
+ "stations": "Garan Settlement,Hoffman Relay,Messerschmid Outpost"
+ },
+ {
+ "name": "Lorden",
+ "pos_x": 13.34375,
+ "pos_y": -84.8125,
+ "pos_z": -4.8125,
+ "stations": "Roentgen Horizons,Haisheng Terminal"
+ },
+ {
+ "name": "Los",
+ "pos_x": -9509.34375,
+ "pos_y": -886.3125,
+ "pos_z": 19820.125,
+ "stations": "Murakami Gateway,Kraken's Retreat"
+ },
+ {
+ "name": "Losin",
+ "pos_x": 1.1875,
+ "pos_y": -29.8125,
+ "pos_z": -35.8125,
+ "stations": "Wohler Landing,Ivanchenkov Hangar"
+ },
+ {
+ "name": "Losna",
+ "pos_x": -6.78125,
+ "pos_y": 79.84375,
+ "pos_z": 52.875,
+ "stations": "Scortia Landing,Rustah Arena,Wagner Platform"
+ },
+ {
+ "name": "Lotia",
+ "pos_x": -65.625,
+ "pos_y": 92.9375,
+ "pos_z": 0.375,
+ "stations": "Bass Horizons,Hudson Base"
+ },
+ {
+ "name": "Lotian",
+ "pos_x": -97.5,
+ "pos_y": 45.5625,
+ "pos_z": 56,
+ "stations": "Wrangell Dock,Arrhenius Station,Buffett Horizons,Rosenberg's Progress,Brust Colony"
+ },
+ {
+ "name": "Lotienets",
+ "pos_x": -43.9375,
+ "pos_y": 112.4375,
+ "pos_z": 1.25,
+ "stations": "Alenquer Station,Steakley Beacon,Wilder Relay,Sturgeon Installation,Vancouver Beacon"
+ },
+ {
+ "name": "Lotigandan",
+ "pos_x": -35.40625,
+ "pos_y": 58.15625,
+ "pos_z": 72.8125,
+ "stations": "Searfoss Point,Schwann Base"
+ },
+ {
+ "name": "Lotigani",
+ "pos_x": -111.78125,
+ "pos_y": 16.84375,
+ "pos_z": 101.1875,
+ "stations": "Kondakova Works"
+ },
+ {
+ "name": "Lotigurara",
+ "pos_x": -41.21875,
+ "pos_y": 100.875,
+ "pos_z": 65.875,
+ "stations": "Ivens Dock,McCaffrey Freeport"
+ },
+ {
+ "name": "Lotileku",
+ "pos_x": 44.15625,
+ "pos_y": -131.78125,
+ "pos_z": -52.59375,
+ "stations": "Wegener Orbital,Skiff Survey"
+ },
+ {
+ "name": "Lougen",
+ "pos_x": 137.53125,
+ "pos_y": -20.875,
+ "pos_z": 5.65625,
+ "stations": "Truly Platform,Trumpler Works,Menzies Bastion"
+ },
+ {
+ "name": "Louguala",
+ "pos_x": -30.84375,
+ "pos_y": 29.15625,
+ "pos_z": 104.71875,
+ "stations": "McMullen City,Akers Port,Brooks Port"
+ },
+ {
+ "name": "Lovae",
+ "pos_x": 27.5625,
+ "pos_y": -95.84375,
+ "pos_z": 138.90625,
+ "stations": "Dickinson Escape,Herschel Settlement"
+ },
+ {
+ "name": "Lovaroju",
+ "pos_x": 94.875,
+ "pos_y": -87.84375,
+ "pos_z": -144.71875,
+ "stations": "Vinge Hub,Williams Landing,Krusvar Enterprise,May Hub"
+ },
+ {
+ "name": "Lovedu",
+ "pos_x": -12.03125,
+ "pos_y": -32.9375,
+ "pos_z": 70.53125,
+ "stations": "Novitski Point,Divis Platform"
+ },
+ {
+ "name": "Lovedui",
+ "pos_x": 97.875,
+ "pos_y": -117.84375,
+ "pos_z": -34.71875,
+ "stations": "Gaspar de Lemos Relay,Hoerner Terminal,Winthrop Landing"
+ },
+ {
+ "name": "Loveri",
+ "pos_x": 88.34375,
+ "pos_y": -212.625,
+ "pos_z": -5.28125,
+ "stations": "Esclangon City,Wallis Bastion,Wetherill Installation"
+ },
+ {
+ "name": "Lowahukula",
+ "pos_x": 88.21875,
+ "pos_y": -47.90625,
+ "pos_z": 133.59375,
+ "stations": "Mattei Colony,Elson Platform,Constantine Beacon"
+ },
+ {
+ "name": "Lowalangi",
+ "pos_x": 169.5625,
+ "pos_y": 48.5625,
+ "pos_z": -25.34375,
+ "stations": "Macan Terminal,Aikin Base,Aguirre Orbital"
+ },
+ {
+ "name": "Lowat Yuan",
+ "pos_x": -14,
+ "pos_y": -20.40625,
+ "pos_z": 84.03125,
+ "stations": "Glenn Port,Tuan Base,Usachov Camp"
+ },
+ {
+ "name": "Lowne 1",
+ "pos_x": -2.8125,
+ "pos_y": -16.59375,
+ "pos_z": -43.40625,
+ "stations": "Sarich Port,Maire's Progress"
+ },
+ {
+ "name": "Lozin",
+ "pos_x": -18.8125,
+ "pos_y": -96.59375,
+ "pos_z": 151.25,
+ "stations": "Lambert Dock,Gwynn Terminal,Silva Relay"
+ },
+ {
+ "name": "LP 1-52",
+ "pos_x": -49.9375,
+ "pos_y": 28.8125,
+ "pos_z": -33.28125,
+ "stations": "Clarke Dock,Garnier Base,Reynolds Penal colony"
+ },
+ {
+ "name": "LP 100-56",
+ "pos_x": -72.6875,
+ "pos_y": 72,
+ "pos_z": -1.46875,
+ "stations": "Wnuk-Lipinski Installation,Williams Installation"
+ },
+ {
+ "name": "LP 101-377",
+ "pos_x": -80.28125,
+ "pos_y": 57.28125,
+ "pos_z": 3.09375,
+ "stations": "Schmidt Depot,Glidden Landing,Sacco City"
+ },
+ {
+ "name": "LP 102-320",
+ "pos_x": -60.625,
+ "pos_y": 35.125,
+ "pos_z": 3.21875,
+ "stations": "Hermaszewski Resort,Ryazanski Station,Mills Platform"
+ },
+ {
+ "name": "LP 104-2",
+ "pos_x": 33.8125,
+ "pos_y": -2.375,
+ "pos_z": 19.84375,
+ "stations": "Jendrassik Installation,Khan Settlement,Fincke Settlement"
+ },
+ {
+ "name": "LP 121-41",
+ "pos_x": -15.59375,
+ "pos_y": 21.0625,
+ "pos_z": -56.875,
+ "stations": "Kennan Co-operative,Christian Vision"
+ },
+ {
+ "name": "LP 128-32",
+ "pos_x": -17.34375,
+ "pos_y": 68.03125,
+ "pos_z": -41.5,
+ "stations": "Bulychev Hub,Irens Point"
+ },
+ {
+ "name": "LP 128-9",
+ "pos_x": -15.71875,
+ "pos_y": 4.21875,
+ "pos_z": -42.3125,
+ "stations": "Fisher Port,Bridges Terminal,Planck Terminal,Lyell Port"
+ },
+ {
+ "name": "LP 131-55",
+ "pos_x": -25.34375,
+ "pos_y": 63.8125,
+ "pos_z": -17.6875,
+ "stations": "Bounds Vision,Abernathy Landing"
+ },
+ {
+ "name": "LP 131-66",
+ "pos_x": -31.5,
+ "pos_y": 71.84375,
+ "pos_z": -20.71875,
+ "stations": "Vasilyev Vision"
+ },
+ {
+ "name": "LP 149-14",
+ "pos_x": -48.3125,
+ "pos_y": -13.40625,
+ "pos_z": -21.53125,
+ "stations": "Levi-Montalcini Terminal,Caidin City,Banks Base"
+ },
+ {
+ "name": "LP 158-2",
+ "pos_x": -39,
+ "pos_y": 8.375,
+ "pos_z": -101.25,
+ "stations": "Redi Enterprise,Lu Park,Niven's Inheritance,Winne Ring,Belyanin Barracks"
+ },
+ {
+ "name": "LP 167-64",
+ "pos_x": -7.34375,
+ "pos_y": 55.78125,
+ "pos_z": -35.59375,
+ "stations": "Alvares Colony,Werber Survey,Sladek Keep"
+ },
+ {
+ "name": "LP 167-71",
+ "pos_x": -11.15625,
+ "pos_y": 61.84375,
+ "pos_z": -39.28125,
+ "stations": "Ziemianski Dock,Herreshoff Dock"
+ },
+ {
+ "name": "LP 176-55",
+ "pos_x": -37.28125,
+ "pos_y": 50.96875,
+ "pos_z": 10.09375,
+ "stations": "Hope Dock,Walker Mines"
+ },
+ {
+ "name": "LP 193-564",
+ "pos_x": -48.40625,
+ "pos_y": -18.96875,
+ "pos_z": -30.8125,
+ "stations": "Gagarin Terminal,Tsibliyev Works"
+ },
+ {
+ "name": "LP 195-25",
+ "pos_x": -79.875,
+ "pos_y": -33.21875,
+ "pos_z": -72.40625,
+ "stations": "Carr Orbital"
+ },
+ {
+ "name": "LP 205-44",
+ "pos_x": -4.125,
+ "pos_y": 8.875,
+ "pos_z": -34.90625,
+ "stations": "Zholobov Vision,Kaku Horizons"
+ },
+ {
+ "name": "LP 211-12",
+ "pos_x": 2.03125,
+ "pos_y": 43.96875,
+ "pos_z": -41.375,
+ "stations": "Dalton Port,Sevastyanov Gateway,Dann Terminal,Shepherd Dock,Lysenko Keep,Joliot-Curie Palace"
+ },
+ {
+ "name": "LP 214-1",
+ "pos_x": 27.4375,
+ "pos_y": -89.96875,
+ "pos_z": 55.875,
+ "stations": "Geston Gateway,Lee City,Hardy Enterprise,Alvarado Survey,Ramsbottom Penal colony"
+ },
+ {
+ "name": "LP 214-26",
+ "pos_x": -2.78125,
+ "pos_y": 85.4375,
+ "pos_z": -39.8125,
+ "stations": "Vardeman Dock"
+ },
+ {
+ "name": "LP 244-47",
+ "pos_x": -55.71875,
+ "pos_y": -28.6875,
+ "pos_z": -3.125,
+ "stations": "Bernard Vision,Bessemer Terminal"
+ },
+ {
+ "name": "LP 245-10",
+ "pos_x": -18.96875,
+ "pos_y": -13.875,
+ "pos_z": -24.28125,
+ "stations": "Galton Gateway,Murdoch Point,Wellman Hub"
+ },
+ {
+ "name": "LP 245-17",
+ "pos_x": -46.90625,
+ "pos_y": -30.3125,
+ "pos_z": -59.4375,
+ "stations": "Hornby Terminal,Arnold City,Bolotov Base"
+ },
+ {
+ "name": "LP 254-26",
+ "pos_x": -1.21875,
+ "pos_y": 14.15625,
+ "pos_z": -49.34375,
+ "stations": "Rey Enterprise,Barba Arsenal,Vela Ring,Reisman Orbital,Harrison Terminal"
+ },
+ {
+ "name": "LP 254-27",
+ "pos_x": -1.5625,
+ "pos_y": 19.65625,
+ "pos_z": -70.9375,
+ "stations": "Reiter Hub"
+ },
+ {
+ "name": "LP 254-40",
+ "pos_x": 1.5625,
+ "pos_y": 23.9375,
+ "pos_z": -86.5625,
+ "stations": "Jacobi Dock"
+ },
+ {
+ "name": "LP 255-11",
+ "pos_x": 1.625,
+ "pos_y": 12.9375,
+ "pos_z": -41.5,
+ "stations": "Burbank Port,Makarov Holdings,Dutton Installation"
+ },
+ {
+ "name": "LP 27-9",
+ "pos_x": -70.40625,
+ "pos_y": 25.4375,
+ "pos_z": -32.40625,
+ "stations": "Curbeam Dock,Drebbel Orbital,Hieb Settlement,McKay Forum,Drebbel Hub,Kwolek Hub,Shonin Orbital"
+ },
+ {
+ "name": "LP 275-83",
+ "pos_x": -58.21875,
+ "pos_y": 70.71875,
+ "pos_z": 37.25,
+ "stations": "Tavares Arsenal,Nusslein-Volhard Terminal,Dozois Beacon"
+ },
+ {
+ "name": "LP 280-9",
+ "pos_x": -56.5,
+ "pos_y": 19.15625,
+ "pos_z": 29.53125,
+ "stations": "Lewitt Colony,Sharp Port,Ivanishin Beacon"
+ },
+ {
+ "name": "LP 282-7",
+ "pos_x": -66.5,
+ "pos_y": 4.15625,
+ "pos_z": 24.3125,
+ "stations": "Herrington Dock,Mukai Gateway,Harrison Town,Zelazny Keep,Reynolds Gateway,Szilard Hub,Bella Port"
+ },
+ {
+ "name": "LP 289-12",
+ "pos_x": -64.46875,
+ "pos_y": -24.40625,
+ "pos_z": -8.1875,
+ "stations": "Vonnegut Terminal"
+ },
+ {
+ "name": "LP 29-188",
+ "pos_x": -67.625,
+ "pos_y": 17.0625,
+ "pos_z": -50.21875,
+ "stations": "Due Holdings,Taylor Enterprise,Warner Works,Pond Dock,Szentmartony Hub"
+ },
+ {
+ "name": "LP 291-34",
+ "pos_x": -47.3125,
+ "pos_y": -21.65625,
+ "pos_z": -18.71875,
+ "stations": "Garratt City,Locke Hub,Doi Dock"
+ },
+ {
+ "name": "LP 292-66",
+ "pos_x": -47.15625,
+ "pos_y": -35.53125,
+ "pos_z": -21.1875,
+ "stations": "Usachov Depot,McClintock Survey,Bulleid Platform"
+ },
+ {
+ "name": "LP 294-40",
+ "pos_x": -51.4375,
+ "pos_y": -38.34375,
+ "pos_z": -37.1875,
+ "stations": "Piaget Hangar,Sadi Carnot Dock,Hogan Terminal"
+ },
+ {
+ "name": "LP 295-2",
+ "pos_x": -77.4375,
+ "pos_y": -56.75,
+ "pos_z": -61.5625,
+ "stations": "Pailes Station,Ross Enterprise,Garriott Ring,Volta Terminal"
+ },
+ {
+ "name": "LP 298-52",
+ "pos_x": -34.65625,
+ "pos_y": -32.78125,
+ "pos_z": -66.15625,
+ "stations": "Fidalgo Terminal,Moore Terminal,Compton Gateway,Wait Colburn Port,Bunsen Legacy,Grigson Vision,Eschbach Station,Brust Port,Selberg Dock,Fernao do Po Hub,Mallory Point"
+ },
+ {
+ "name": "LP 30-55",
+ "pos_x": -26.5625,
+ "pos_y": 6.75,
+ "pos_z": -20.71875,
+ "stations": "Crown Platform,Abasheli Stop"
+ },
+ {
+ "name": "LP 302-22",
+ "pos_x": -18.875,
+ "pos_y": -17.0625,
+ "pos_z": -79.6875,
+ "stations": "Bloomfield Dock,Parise Hub,Avicenna City,Carrier Dock,Boming Terminal,Ramsay Station,Clement Dock,Keyes Landing,Wolf Port,Asaro Laboratory,Fermi Orbital,Brand Keep,Cobb's Progress"
+ },
+ {
+ "name": "LP 308-10",
+ "pos_x": 4.78125,
+ "pos_y": 16.5625,
+ "pos_z": -58.375,
+ "stations": "Wegener Plant,Pettit Hub"
+ },
+ {
+ "name": "LP 320-359",
+ "pos_x": 1.25,
+ "pos_y": 49.71875,
+ "pos_z": -6.5625,
+ "stations": "McMullen Point,Bondar Port,Vaugh Orbital,Wagner Gateway,Franklin Terminal,Pauling Port,Wolf Orbital"
+ },
+ {
+ "name": "LP 322-836",
+ "pos_x": -3.4375,
+ "pos_y": 51.59375,
+ "pos_z": 2.03125,
+ "stations": "Fife Platform,Clairaut Terminal,Peral Reach,Gaspar de Portola Dock,Kronecker Terminal"
+ },
+ {
+ "name": "LP 329-20",
+ "pos_x": -36.4375,
+ "pos_y": 54.21875,
+ "pos_z": 31.375,
+ "stations": "Guest Hangar,Flade Refinery"
+ },
+ {
+ "name": "LP 332-45",
+ "pos_x": -57.40625,
+ "pos_y": 36.53125,
+ "pos_z": 44.71875,
+ "stations": "Watson Enterprise,Teng Beacon,Fawcett Oasis,Thoreau Station"
+ },
+ {
+ "name": "LP 336-4",
+ "pos_x": -35.5,
+ "pos_y": 7.53125,
+ "pos_z": 17.4375,
+ "stations": "Bolotov City,Mendez Orbital,Varley Silo"
+ },
+ {
+ "name": "LP 336-6",
+ "pos_x": -23.46875,
+ "pos_y": 5.09375,
+ "pos_z": 11.4375,
+ "stations": "Lu Installation,Nusslein-Volhard Installation,Crown Relay"
+ },
+ {
+ "name": "LP 336-71",
+ "pos_x": 41.875,
+ "pos_y": 7.25,
+ "pos_z": 77.65625,
+ "stations": "Garriott Gateway"
+ },
+ {
+ "name": "LP 339-7",
+ "pos_x": -70.0625,
+ "pos_y": -8.28125,
+ "pos_z": 27.90625,
+ "stations": "Whitson Orbital,So-yeon Dock,Gell-Mann Ring,Scott Beacon,Earth's Inheritance Wildlife Reserve,Chapman Hub,Lazarev Enterprise,Thirsk Survey,Kerwin Gateway,Potter Horizons"
+ },
+ {
+ "name": "LP 340-547",
+ "pos_x": -53.34375,
+ "pos_y": -8.28125,
+ "pos_z": 11.90625,
+ "stations": "Appel Terminal,Pauli Orbital"
+ },
+ {
+ "name": "LP 347-5",
+ "pos_x": -49.53125,
+ "pos_y": -27.6875,
+ "pos_z": -14.96875,
+ "stations": "Bernard City,Bohr Hub,Alvarado Laboratory,Mohmand Terminal,Boulton City,Hertz City,Davis Terminal,Arnarson Silo,Jun Dock,Cockrell City,Gresley Dock"
+ },
+ {
+ "name": "LP 348-64",
+ "pos_x": -121.3125,
+ "pos_y": -101.46875,
+ "pos_z": -54,
+ "stations": "Chios Camp"
+ },
+ {
+ "name": "LP 349-61",
+ "pos_x": -61.9375,
+ "pos_y": -60.5625,
+ "pos_z": -37.46875,
+ "stations": "McDevitt Hub,Aikin Ring,Peary Co-operative,Payne Station,Cook Bastion,Bartlett Survey"
+ },
+ {
+ "name": "LP 350-75",
+ "pos_x": -61.1875,
+ "pos_y": -56.625,
+ "pos_z": -46.65625,
+ "stations": "Butler Dock,Wilhelm Hub,Brule Co-operative,Dedekind Laboratory,MacCurdy Silo"
+ },
+ {
+ "name": "LP 353-74",
+ "pos_x": -37.59375,
+ "pos_y": -44.75,
+ "pos_z": -59.84375,
+ "stations": "Holland Depot,Oersted Mines,Zewail Mine"
+ },
+ {
+ "name": "LP 355-51",
+ "pos_x": -21,
+ "pos_y": -31.25,
+ "pos_z": -59.59375,
+ "stations": "Cousteau Platform,H. G. Wells Point"
+ },
+ {
+ "name": "LP 355-65",
+ "pos_x": -22.6875,
+ "pos_y": -30.28125,
+ "pos_z": -61.84375,
+ "stations": "Barba Ring,Miklouho-Maclay Silo,Boulton Port,Vinogradov Enterprise,Sheffield Camp"
+ },
+ {
+ "name": "LP 356-106",
+ "pos_x": -17.1875,
+ "pos_y": -24.5,
+ "pos_z": -51.21875,
+ "stations": "Carrier Dock"
+ },
+ {
+ "name": "LP 36-115",
+ "pos_x": -41.6875,
+ "pos_y": 46,
+ "pos_z": -49.78125,
+ "stations": "Merbold Holdings,Manakov Settlement"
+ },
+ {
+ "name": "LP 37-75",
+ "pos_x": -27.25,
+ "pos_y": 38.5,
+ "pos_z": -33.65625,
+ "stations": "Shepard Beacon,Barr Silo,Linenger Dock"
+ },
+ {
+ "name": "LP 375-23",
+ "pos_x": 20.25,
+ "pos_y": 101.21875,
+ "pos_z": -19.9375,
+ "stations": "Horowitz Terminal,Vinci Terminal,Bordage Terminal,Markham Base"
+ },
+ {
+ "name": "LP 375-25",
+ "pos_x": 9.53125,
+ "pos_y": 59.3125,
+ "pos_z": -13.21875,
+ "stations": "Slonczewski Vision,King Gateway,Lorrah Hub"
+ },
+ {
+ "name": "LP 376-62",
+ "pos_x": 9.15625,
+ "pos_y": 83,
+ "pos_z": -4.59375,
+ "stations": "Mallory Relay,Reed Terminal"
+ },
+ {
+ "name": "LP 377-100",
+ "pos_x": 7.5625,
+ "pos_y": 70.4375,
+ "pos_z": -2.5625,
+ "stations": "Hutchinson Orbital,Levy Survey,Phillips Port,Hill Ring"
+ },
+ {
+ "name": "LP 377-78",
+ "pos_x": 5.96875,
+ "pos_y": 81.21875,
+ "pos_z": 3.875,
+ "stations": "Key Dock,Beltrami City,Jones Hub,Gaspar de Lemos Port,Janifer Port,Reynolds Orbital,Macedo Horizons,Cochrane City,Heinlein Keep,Napier Port,Semeonis Terminal"
+ },
+ {
+ "name": "LP 385-58",
+ "pos_x": -36.90625,
+ "pos_y": 54.21875,
+ "pos_z": 41.5625,
+ "stations": "Holden Installation,Elvstrom Dock"
+ },
+ {
+ "name": "LP 387-31",
+ "pos_x": -49.625,
+ "pos_y": 47.65625,
+ "pos_z": 47.53125,
+ "stations": "Beadle Relay,Rasmussen Platform"
+ },
+ {
+ "name": "LP 390-16",
+ "pos_x": -32.3125,
+ "pos_y": 14.28125,
+ "pos_z": 24.375,
+ "stations": "Readdy Orbital,Fontana Horizons,Payton Hub,Bluford Vision"
+ },
+ {
+ "name": "LP 399-165",
+ "pos_x": -61.40625,
+ "pos_y": -28.4375,
+ "pos_z": 6.5,
+ "stations": "Tombaugh City,McDaniel Laboratory"
+ },
+ {
+ "name": "LP 403-71",
+ "pos_x": -108.09375,
+ "pos_y": -95.125,
+ "pos_z": -36.5,
+ "stations": "Brunton Works,Tereshkova Dock"
+ },
+ {
+ "name": "LP 404-6",
+ "pos_x": -42.8125,
+ "pos_y": -39.1875,
+ "pos_z": -12.3125,
+ "stations": "Thornton Terminal,Vizcaino Depot,Jenner Hub,Chaudhary Dock"
+ },
+ {
+ "name": "LP 404-66",
+ "pos_x": -49.90625,
+ "pos_y": -48.59375,
+ "pos_z": -20.15625,
+ "stations": "Morgan Stop"
+ },
+ {
+ "name": "LP 410-81",
+ "pos_x": -33.125,
+ "pos_y": -63.84375,
+ "pos_z": -78.9375,
+ "stations": "Asire Port,Borel Dock"
+ },
+ {
+ "name": "LP 410-93",
+ "pos_x": -15.59375,
+ "pos_y": -25.96875,
+ "pos_z": -32.4375,
+ "stations": "Foucault Ring,Oluwafemi Hub,Fossum Base,Veach Orbital,Van Scyoc Relay"
+ },
+ {
+ "name": "LP 415-1446",
+ "pos_x": -0.53125,
+ "pos_y": -30.90625,
+ "pos_z": -71.28125,
+ "stations": "Bardeen Plant"
+ },
+ {
+ "name": "LP 417-213",
+ "pos_x": 9.4375,
+ "pos_y": -9.5,
+ "pos_z": -55.125,
+ "stations": "Gibson Survey,Liouville Base,Clark Hub,Camarda Hub,Gillekens Hub,Vernadsky Dock,Knipling Orbital,Creighton Works,Bacon Station,Zetford Works,Cleave Settlement,Richards Settlement"
+ },
+ {
+ "name": "LP 421-7",
+ "pos_x": 17.4375,
+ "pos_y": 9.03125,
+ "pos_z": -58.34375,
+ "stations": "Cardano Horizons"
+ },
+ {
+ "name": "LP 424-4",
+ "pos_x": 31.125,
+ "pos_y": 28.09375,
+ "pos_z": -63.21875,
+ "stations": "Matteucci Settlement"
+ },
+ {
+ "name": "LP 431-71",
+ "pos_x": 47.59375,
+ "pos_y": 120.03125,
+ "pos_z": -38.21875,
+ "stations": "Remec Dock,Harris Colony"
+ },
+ {
+ "name": "LP 440-38",
+ "pos_x": -4.65625,
+ "pos_y": 43.78125,
+ "pos_z": 20.6875,
+ "stations": "Skvortsov Settlement"
+ },
+ {
+ "name": "LP 442-37",
+ "pos_x": -29.65625,
+ "pos_y": 88.8125,
+ "pos_z": 57.65625,
+ "stations": "Bryant Hub,Cleve City"
+ },
+ {
+ "name": "LP 445-22",
+ "pos_x": -32.5625,
+ "pos_y": 49.65625,
+ "pos_z": 52.90625,
+ "stations": "Parry Survey,Lyakhov Bastion,Trevithick Horizons"
+ },
+ {
+ "name": "LP 447-38",
+ "pos_x": -34.6875,
+ "pos_y": 29.28125,
+ "pos_z": 41.53125,
+ "stations": "von Krusenstern Hub"
+ },
+ {
+ "name": "LP 448-41",
+ "pos_x": -35.96875,
+ "pos_y": 19.875,
+ "pos_z": 39.8125,
+ "stations": "Hopkins Landing,Watson Arena,Bhabha Refinery"
+ },
+ {
+ "name": "LP 45-128",
+ "pos_x": -69.28125,
+ "pos_y": 32.25,
+ "pos_z": -13.90625,
+ "stations": "Khrenov Terminal,Stein City,Marusek Hub,Robinson Vision"
+ },
+ {
+ "name": "LP 455-12",
+ "pos_x": -46.5,
+ "pos_y": -10.9375,
+ "pos_z": 29.71875,
+ "stations": "Bernoulli Gateway,Gamow Station,Dunn Terminal"
+ },
+ {
+ "name": "LP 458-64",
+ "pos_x": -36.1875,
+ "pos_y": -16.46875,
+ "pos_z": 10,
+ "stations": "Aleksandrov Port,Drexler Orbital,Binnie Horizons"
+ },
+ {
+ "name": "LP 460-60",
+ "pos_x": -60.0625,
+ "pos_y": -38.65625,
+ "pos_z": 7.5,
+ "stations": "Acton Depot,Julian Enterprise,Liska Terminal,Wegener Orbital,Burnell Base"
+ },
+ {
+ "name": "LP 462-13",
+ "pos_x": -78.75,
+ "pos_y": -61.625,
+ "pos_z": -7,
+ "stations": "Vonnegut Bastion,Glashow Colony,Birkeland Installation,Cugnot Hub,Haberlandt Settlement"
+ },
+ {
+ "name": "LP 462-19",
+ "pos_x": -32.875,
+ "pos_y": -25.75,
+ "pos_z": -2.59375,
+ "stations": "Tarelkin Dock,Hoard Base,Shaw Arsenal"
+ },
+ {
+ "name": "LP 465-14",
+ "pos_x": 75.75,
+ "pos_y": 26.03125,
+ "pos_z": 5.15625,
+ "stations": "Kanwar Dock,White Station,Alten Plant,Cori Ring,Dunbar Relay"
+ },
+ {
+ "name": "LP 466-235",
+ "pos_x": -38,
+ "pos_y": -52.84375,
+ "pos_z": -31.09375,
+ "stations": "Joliot-Curie Port,Locke Terminal,Crippen Hub,Linnaeus Depot,Altshuller Base"
+ },
+ {
+ "name": "LP 470-30",
+ "pos_x": -25.125,
+ "pos_y": -55,
+ "pos_z": -57.84375,
+ "stations": "Bayley Installation,Curie Horizons"
+ },
+ {
+ "name": "LP 470-65",
+ "pos_x": 82.9375,
+ "pos_y": 39.5625,
+ "pos_z": 41.1875,
+ "stations": "Schrodinger City,Hawley Ring"
+ },
+ {
+ "name": "LP 476-207",
+ "pos_x": 18.6875,
+ "pos_y": -32.8125,
+ "pos_z": -97.5,
+ "stations": "Gromov Terminal,North Penal colony,Cook Terminal,Gunn Prospect,Gerlache Orbital,Nelder Terminal,Stapledon Hub,Clark Dock,Kopra Point"
+ },
+ {
+ "name": "LP 48-567",
+ "pos_x": -67.0625,
+ "pos_y": 14.96875,
+ "pos_z": -25.5625,
+ "stations": "Noakes Hangar,Crown Holdings,Robinson's Inheritance,Anders Beacon"
+ },
+ {
+ "name": "LP 486-49",
+ "pos_x": 31.40625,
+ "pos_y": 38,
+ "pos_z": -42.6875,
+ "stations": "Fisher's Progress,Napier Enterprise,Cortes Hub,Sucharitkul Port,Coney Installation,Litke Station,Ingstad Gateway,Bering Point"
+ },
+ {
+ "name": "LP 490-68",
+ "pos_x": 56.75,
+ "pos_y": 88.59375,
+ "pos_z": -37.25,
+ "stations": "Shaara Ring,Woolley Barracks,Menezes Gateway,Varley Hub"
+ },
+ {
+ "name": "LP 493-64",
+ "pos_x": 19.1875,
+ "pos_y": 47.96875,
+ "pos_z": -3.0625,
+ "stations": "Chern Settlement,Ramanujan Hub,Fossey Gateway"
+ },
+ {
+ "name": "LP 499-54",
+ "pos_x": 1.96875,
+ "pos_y": 68.28125,
+ "pos_z": 32.9375,
+ "stations": "Precourt City,Fozard Beacon,Revin Ring,Porges Depot,Poleshchuk Installation"
+ },
+ {
+ "name": "LP 499-59",
+ "pos_x": 1.03125,
+ "pos_y": 53.25,
+ "pos_z": 26.03125,
+ "stations": "Sanger Vision"
+ },
+ {
+ "name": "LP 5-88",
+ "pos_x": -27.28125,
+ "pos_y": 19.59375,
+ "pos_z": -23.03125,
+ "stations": "Hirase Holdings,Song Dock,Campbell Relay"
+ },
+ {
+ "name": "LP 51-17",
+ "pos_x": -60.0625,
+ "pos_y": 5.15625,
+ "pos_z": -40.28125,
+ "stations": "Macquorn Rankine Dock,Burnet Ring,Virchow Landing,Lavrador Arsenal"
+ },
+ {
+ "name": "LP 510-10",
+ "pos_x": -39.90625,
+ "pos_y": 11.59375,
+ "pos_z": 46.96875,
+ "stations": "Born Horizons,White Depot,Bokeili Relay"
+ },
+ {
+ "name": "LP 525-39",
+ "pos_x": -19.71875,
+ "pos_y": -31.125,
+ "pos_z": -9.09375,
+ "stations": "Fuglesang Depot,Clark Installation,Atwater Outpost"
+ },
+ {
+ "name": "LP 532-81",
+ "pos_x": -1.5625,
+ "pos_y": -27.375,
+ "pos_z": -32.3125,
+ "stations": "Savitskaya Refinery,Pytheas' Inheritance,Marques Survey,Hieb City"
+ },
+ {
+ "name": "LP 535-11",
+ "pos_x": 11.5,
+ "pos_y": -37.09375,
+ "pos_z": -65.1875,
+ "stations": "Moisuc Hub"
+ },
+ {
+ "name": "LP 542-33",
+ "pos_x": 29.15625,
+ "pos_y": 15.65625,
+ "pos_z": -44.75,
+ "stations": "Reynolds Holdings,Doi Station"
+ },
+ {
+ "name": "LP 547-159",
+ "pos_x": 55.4375,
+ "pos_y": 38.28125,
+ "pos_z": 56.1875,
+ "stations": "Martin Gateway,Tarski Relay,Weiss Dock,Dedman Port"
+ },
+ {
+ "name": "LP 552-48",
+ "pos_x": 48.46875,
+ "pos_y": 91.875,
+ "pos_z": -13.71875,
+ "stations": "Virtanen Terminal,Anning Port"
+ },
+ {
+ "name": "LP 560-66",
+ "pos_x": -0.09375,
+ "pos_y": 75.1875,
+ "pos_z": 50.5625,
+ "stations": "Berners-Lee Survey,Lazarev Outpost"
+ },
+ {
+ "name": "LP 564-39",
+ "pos_x": -17.9375,
+ "pos_y": 47.625,
+ "pos_z": 56.21875,
+ "stations": "Kidman City,Hilbert Landing,Euclid Penal colony"
+ },
+ {
+ "name": "LP 575-38",
+ "pos_x": -54.03125,
+ "pos_y": -19.40625,
+ "pos_z": 44.25,
+ "stations": "Jenner Port,McCulley City,Hutton Port,Alenquer Lab,Acton Base"
+ },
+ {
+ "name": "LP 576-34",
+ "pos_x": -58.90625,
+ "pos_y": -37.6875,
+ "pos_z": 41.8125,
+ "stations": "Pavlov Plant,Curbeam Hangar,Wood Vision"
+ },
+ {
+ "name": "LP 58-247",
+ "pos_x": -24.25,
+ "pos_y": 28.53125,
+ "pos_z": -46,
+ "stations": "Brand Colony"
+ },
+ {
+ "name": "LP 580-33",
+ "pos_x": -48.8125,
+ "pos_y": -50.9375,
+ "pos_z": 16.75,
+ "stations": "Shkaplerov Ring,Fraley Enterprise,Tyurin Dock,Weil Installation,Lorenz Installation"
+ },
+ {
+ "name": "LP 581-36",
+ "pos_x": -53.40625,
+ "pos_y": -58.40625,
+ "pos_z": 10.125,
+ "stations": "Margulis Gateway,Musgrave Gateway,Grover Port,McKay Gateway,Acton Dock,Atkov Ring,Coulter Beacon,Cartier Relay,Rescue Ship - Acton Dock"
+ },
+ {
+ "name": "LP 583-82",
+ "pos_x": -45.96875,
+ "pos_y": -59.875,
+ "pos_z": -7.75,
+ "stations": "Wells Gateway,Taylor Dock,Griffin Installation"
+ },
+ {
+ "name": "LP 590-137",
+ "pos_x": -12.15625,
+ "pos_y": -56.8125,
+ "pos_z": -43.09375,
+ "stations": "Lanchester Settlement,Archimedes Colony,Verne Beacon"
+ },
+ {
+ "name": "LP 60-205",
+ "pos_x": -31.96875,
+ "pos_y": 46.84375,
+ "pos_z": -50.375,
+ "stations": "Kneale Ring"
+ },
+ {
+ "name": "LP 617-37",
+ "pos_x": 22.28125,
+ "pos_y": 58.59375,
+ "pos_z": 25.5,
+ "stations": "Meyrink Oasis,Worlidge Station,Levchenko Horizons"
+ },
+ {
+ "name": "LP 620-3",
+ "pos_x": 8.875,
+ "pos_y": 44.96875,
+ "pos_z": 30.875,
+ "stations": "Jensen Enterprise,Shkaplerov City,Gresh Penal colony"
+ },
+ {
+ "name": "LP 621-11",
+ "pos_x": 7.15625,
+ "pos_y": 48.5,
+ "pos_z": 42.03125,
+ "stations": "Horch Orbital,Kettle Bastion"
+ },
+ {
+ "name": "LP 634-24",
+ "pos_x": -66.6875,
+ "pos_y": -35.75,
+ "pos_z": 71.125,
+ "stations": "Yamazaki Terminal,Rzeppa Relay,Garcia Prospect"
+ },
+ {
+ "name": "LP 635-46",
+ "pos_x": -59.28125,
+ "pos_y": -38.34375,
+ "pos_z": 55.34375,
+ "stations": "Lyakhov Settlement,Cleave Plant"
+ },
+ {
+ "name": "LP 638-58",
+ "pos_x": -40.75,
+ "pos_y": -41.375,
+ "pos_z": 26.84375,
+ "stations": "Harrison Settlement,Coblentz City,Benford Survey,Rond d'Alembert Port,Thorne's Inheritance"
+ },
+ {
+ "name": "LP 64-194",
+ "pos_x": -21.65625,
+ "pos_y": 32.21875,
+ "pos_z": -16.21875,
+ "stations": "Longyear Survey,Nehsi Prospect"
+ },
+ {
+ "name": "LP 640-74",
+ "pos_x": -45.1875,
+ "pos_y": -49.65625,
+ "pos_z": 18.15625,
+ "stations": "Phillips Arsenal,Merle Installation,Guerrero Hub"
+ },
+ {
+ "name": "LP 641-4",
+ "pos_x": -54.90625,
+ "pos_y": -65.03125,
+ "pos_z": 17.34375,
+ "stations": "Reiter Point,Siemens Port,Makeev Bastion"
+ },
+ {
+ "name": "LP 644-15",
+ "pos_x": -64.125,
+ "pos_y": -131.3125,
+ "pos_z": -4.90625,
+ "stations": "Alasdair's Orbital,Lupoff Dock,Besonders Landing"
+ },
+ {
+ "name": "LP 658-33",
+ "pos_x": 25.15625,
+ "pos_y": -14.96875,
+ "pos_z": -43.21875,
+ "stations": "Hawley Survey,Cernan Prospect,Porsche Settlement,Vian Landing"
+ },
+ {
+ "name": "LP 672-4",
+ "pos_x": 59.3125,
+ "pos_y": 71.1875,
+ "pos_z": -8.125,
+ "stations": "Newton City"
+ },
+ {
+ "name": "LP 672-42",
+ "pos_x": 27.78125,
+ "pos_y": 32.84375,
+ "pos_z": 1,
+ "stations": "Conti Ring,Piercy City,Griffith Dock,Hartog Escape,Elder Oasis"
+ },
+ {
+ "name": "LP 673-13",
+ "pos_x": 33.15625,
+ "pos_y": 43.03125,
+ "pos_z": 0.40625,
+ "stations": "Hobaugh Prospect,Henry Orbital,Murray Port,Besonders Depot,Gurney Station,Atwater Orbital,Frimout Dock"
+ },
+ {
+ "name": "LP 673-42",
+ "pos_x": 40.03125,
+ "pos_y": 53.34375,
+ "pos_z": 6.90625,
+ "stations": "Dirichlet Freeport"
+ },
+ {
+ "name": "LP 675-76",
+ "pos_x": 27.21875,
+ "pos_y": 45.59375,
+ "pos_z": 11.59375,
+ "stations": "Pinto Observatory,Andrews Arena"
+ },
+ {
+ "name": "LP 681-91",
+ "pos_x": 9.5,
+ "pos_y": 55.65625,
+ "pos_z": 56.53125,
+ "stations": "Hertz Dock,Behnken Refinery"
+ },
+ {
+ "name": "LP 685-43",
+ "pos_x": -13.40625,
+ "pos_y": 32.46875,
+ "pos_z": 67.1875,
+ "stations": "Metz Vision,Cartan Gateway,Lie Ring"
+ },
+ {
+ "name": "LP 69-457",
+ "pos_x": -50.59375,
+ "pos_y": 45.65625,
+ "pos_z": 24.65625,
+ "stations": "Carrier Hub,Liska Platform"
+ },
+ {
+ "name": "LP 694-16",
+ "pos_x": -28.5,
+ "pos_y": -18.4375,
+ "pos_z": 42.46875,
+ "stations": "Chapman Terminal"
+ },
+ {
+ "name": "LP 7-226",
+ "pos_x": -42.78125,
+ "pos_y": 31.75,
+ "pos_z": -27.25,
+ "stations": "Strzelecki Gateway"
+ },
+ {
+ "name": "LP 701-7",
+ "pos_x": -31.53125,
+ "pos_y": -49.125,
+ "pos_z": 16.875,
+ "stations": "Coulomb Station,Hirase Depot,Creighton Hub,Dutton Laboratory,Rominger Gateway,Ibold Hub"
+ },
+ {
+ "name": "LP 708-253",
+ "pos_x": -7.9375,
+ "pos_y": -108.28125,
+ "pos_z": -34.28125,
+ "stations": "Gibson Platform"
+ },
+ {
+ "name": "LP 71-157",
+ "pos_x": -52.34375,
+ "pos_y": 28.375,
+ "pos_z": -8.03125,
+ "stations": "Carson Port,Levi-Montalcini Platform"
+ },
+ {
+ "name": "LP 711-32",
+ "pos_x": 9.21875,
+ "pos_y": -60.90625,
+ "pos_z": -38.25,
+ "stations": "Beliaev City,Nylund Station,Panshin Gateway,Bear Terminal,Block Terminal,Lloyd Landing,Buchli Holdings,Henson Enterprise"
+ },
+ {
+ "name": "LP 711-43",
+ "pos_x": 9.65625,
+ "pos_y": -56.3125,
+ "pos_z": -36.8125,
+ "stations": "Spinrad Gateway,McCarthy Orbital"
+ },
+ {
+ "name": "LP 714-58",
+ "pos_x": 24.21875,
+ "pos_y": -44.21875,
+ "pos_z": -48.09375,
+ "stations": "Ahmed Bastion,Macquorn Rankine Depot,Mondeh Outpost"
+ },
+ {
+ "name": "LP 715-52",
+ "pos_x": 13.96875,
+ "pos_y": -20.3125,
+ "pos_z": -26.53125,
+ "stations": "Melnick Ring,Pauli Installation"
+ },
+ {
+ "name": "LP 716-35",
+ "pos_x": 28,
+ "pos_y": -29.25,
+ "pos_z": -44.84375,
+ "stations": "Hahn Settlement,Tyurin Hangar,Conway Installation,Gibson Arsenal"
+ },
+ {
+ "name": "LP 718-47",
+ "pos_x": 48.875,
+ "pos_y": -27.65625,
+ "pos_z": -66.75,
+ "stations": "Lucretius City,Curie Holdings,Roberts Orbital"
+ },
+ {
+ "name": "LP 726-6",
+ "pos_x": 56.8125,
+ "pos_y": 24.03125,
+ "pos_z": -38.03125,
+ "stations": "Merbold Station,Vesalius Gateway,Kirk's Inheritance,Grabe Hub,Deb Arsenal"
+ },
+ {
+ "name": "LP 732-94",
+ "pos_x": 33.28125,
+ "pos_y": 32.34375,
+ "pos_z": 1.03125,
+ "stations": "Gutierrez Installation,Kubasov Ring,Vetulani Station,Gemar Port,Conway Base"
+ },
+ {
+ "name": "LP 734-11",
+ "pos_x": 36.9375,
+ "pos_y": 41.28125,
+ "pos_z": 9.625,
+ "stations": "Rothman Freeport,Rosenberg Dock,Napier Freeport"
+ },
+ {
+ "name": "LP 734-32",
+ "pos_x": 18.96875,
+ "pos_y": 21.15625,
+ "pos_z": 6.28125,
+ "stations": "Franklin Ring,Green Enterprise,Sargent Arsenal"
+ },
+ {
+ "name": "LP 744-46",
+ "pos_x": 4.25,
+ "pos_y": 45.9375,
+ "pos_z": 90.09375,
+ "stations": "Galindo Port,Serrao Dock,Crouch Lab,Jacobi Co-operative"
+ },
+ {
+ "name": "LP 747-25",
+ "pos_x": -14.0625,
+ "pos_y": 17.21875,
+ "pos_z": 84.875,
+ "stations": "Wells Dock,Holland Dock"
+ },
+ {
+ "name": "LP 751-1",
+ "pos_x": -22.84375,
+ "pos_y": -9.34375,
+ "pos_z": 56.25,
+ "stations": "Carey Hub,Bowen Silo,Tito Orbital,Jenner Enterprise,Lamarr Ring,Bamford Port,Ramsbottom Landing,Grissom Orbital"
+ },
+ {
+ "name": "LP 762-3",
+ "pos_x": -24.5625,
+ "pos_y": -58.375,
+ "pos_z": 15.71875,
+ "stations": "McCoy Landing,Lucretius Port,Houssay Outpost"
+ },
+ {
+ "name": "LP 764-108",
+ "pos_x": -16.125,
+ "pos_y": -73.9375,
+ "pos_z": 0.34375,
+ "stations": "Meikle Works,von Helmont Point,May Silo"
+ },
+ {
+ "name": "LP 764-39",
+ "pos_x": -27.34375,
+ "pos_y": -104.875,
+ "pos_z": 9.5,
+ "stations": "Oosterhoff Mine"
+ },
+ {
+ "name": "LP 771-72",
+ "pos_x": 13.46875,
+ "pos_y": -54.15625,
+ "pos_z": -31.09375,
+ "stations": "Levchenko Installation,Scheutz Enterprise,Vernadsky Platform"
+ },
+ {
+ "name": "LP 772-72",
+ "pos_x": 18.75,
+ "pos_y": -59.65625,
+ "pos_z": -42.96875,
+ "stations": "Tilley Relay"
+ },
+ {
+ "name": "LP 787-52",
+ "pos_x": 35.40625,
+ "pos_y": 14.375,
+ "pos_z": -14.21875,
+ "stations": "Tanner Orbital,Ewald Enterprise,Cassidy Arsenal,Boulle Landing,Scully-Power Port"
+ },
+ {
+ "name": "LP 790-29",
+ "pos_x": 39.78125,
+ "pos_y": 25.34375,
+ "pos_z": -3.03125,
+ "stations": "Thagard Point,Rominger City,Metcalf Plant"
+ },
+ {
+ "name": "LP 792-20",
+ "pos_x": 41.53125,
+ "pos_y": 34.78125,
+ "pos_z": 4,
+ "stations": "Wakata Landing,Lorenz Platform,Wood Depot"
+ },
+ {
+ "name": "LP 792-33",
+ "pos_x": 54.8125,
+ "pos_y": 49.65625,
+ "pos_z": 7.71875,
+ "stations": "Borlaug Port,Kotov Station,Hart City"
+ },
+ {
+ "name": "LP 798-44",
+ "pos_x": 33.3125,
+ "pos_y": 42.875,
+ "pos_z": 40.8125,
+ "stations": "Resnick Installation,Evangelisti Survey,Slusser Colony,Forstchen Dock,Abel Relay"
+ },
+ {
+ "name": "LP 800-6",
+ "pos_x": 29.84375,
+ "pos_y": 47.15625,
+ "pos_z": 53.96875,
+ "stations": "Hadfield Colony,Key's Inheritance,Precourt Hub"
+ },
+ {
+ "name": "LP 802-68",
+ "pos_x": 20.1875,
+ "pos_y": 59.125,
+ "pos_z": 93.875,
+ "stations": "Swigert Port,Pausch Enterprise,Cleave Dock,Anders Barracks"
+ },
+ {
+ "name": "LP 802-69",
+ "pos_x": 13.8125,
+ "pos_y": 41.5,
+ "pos_z": 68.375,
+ "stations": "Yu Station,Divis Bastion,Ostrander Plant"
+ },
+ {
+ "name": "LP 804-27",
+ "pos_x": 3.3125,
+ "pos_y": 17.84375,
+ "pos_z": 43.28125,
+ "stations": "Lupoff Vista,Wrangell Terminal,Poleshchuk Landing,Cogswell Hub,Tyurin Terminal"
+ },
+ {
+ "name": "LP 806-8",
+ "pos_x": -3.90625,
+ "pos_y": 18,
+ "pos_z": 56.5,
+ "stations": "Creighton Vision,Gerst Oasis,Bayliss' Inheritance"
+ },
+ {
+ "name": "LP 811-17",
+ "pos_x": -22.15625,
+ "pos_y": -14.125,
+ "pos_z": 73.09375,
+ "stations": "Shalatula Colony,Doyle Dock,Harper Dock,Grego Barracks"
+ },
+ {
+ "name": "LP 816-60",
+ "pos_x": -7.8125,
+ "pos_y": -10.65625,
+ "pos_z": 13.15625,
+ "stations": "Garratt Landing,Moore Platform,Kennan Bastion"
+ },
+ {
+ "name": "LP 816-61",
+ "pos_x": -47.84375,
+ "pos_y": -64.5625,
+ "pos_z": 75.3125,
+ "stations": "Garneau Holdings"
+ },
+ {
+ "name": "LP 825-559",
+ "pos_x": -6.40625,
+ "pos_y": -52.21875,
+ "pos_z": -0.59375,
+ "stations": "Pinto Station,Larson Terminal,Katzenstein Terminal,Hopkinson Terminal,Malpighi Keep,Griffin Beacon"
+ },
+ {
+ "name": "LP 834-42",
+ "pos_x": 30.53125,
+ "pos_y": -39.40625,
+ "pos_z": -32.15625,
+ "stations": "Moisuc Dock,Kekule Station,Nusslein-Volhard Base,Galvani Station,Tani Relay"
+ },
+ {
+ "name": "LP 838-16",
+ "pos_x": 27.5,
+ "pos_y": -12.71875,
+ "pos_z": -21.25,
+ "stations": "Tenn Port,Lawhead Hub,Chalker Ring,Panshin Market,Walker Vista"
+ },
+ {
+ "name": "LP 84-34",
+ "pos_x": -45.90625,
+ "pos_y": 13.3125,
+ "pos_z": -75.21875,
+ "stations": "Bertin Colony,Qureshi Installation,Rolland Outpost"
+ },
+ {
+ "name": "LP 840-15",
+ "pos_x": 45.46875,
+ "pos_y": -5.78125,
+ "pos_z": -32.875,
+ "stations": "McCulley Terminal,Treshchov Port,Hire Landing,Noriega Terminal,Kimberlin Relay"
+ },
+ {
+ "name": "LP 844-28",
+ "pos_x": 36.125,
+ "pos_y": 9.3125,
+ "pos_z": -13.75,
+ "stations": "Reed Depot,Shepard Enterprise,Anders Station"
+ },
+ {
+ "name": "LP 847-48",
+ "pos_x": 34.0625,
+ "pos_y": 13.375,
+ "pos_z": -4.78125,
+ "stations": "Rozhdestvensky Hub,Planck Terminal,Anderson Installation,Battuta City,Celsius Port"
+ },
+ {
+ "name": "LP 849-20",
+ "pos_x": 78.71875,
+ "pos_y": 50.21875,
+ "pos_z": 1.0625,
+ "stations": "Divis Port,Hoshide Installation,Mattingly Terminal,Matteucci City,Bardeen Dock,Crown Hub,Galilei Enterprise,Bacon Point,Vernadsky Terminal,Kekule Depot,Becquerel Dock"
+ },
+ {
+ "name": "LP 855-34",
+ "pos_x": 33.625,
+ "pos_y": 33.8125,
+ "pos_z": 33.375,
+ "stations": "Acton Port,Covey Point"
+ },
+ {
+ "name": "LP 861-12",
+ "pos_x": 12.3125,
+ "pos_y": 23.1875,
+ "pos_z": 61.125,
+ "stations": "Artyukhin Terminal,Thoreau Ring,Klimuk Depot"
+ },
+ {
+ "name": "LP 862-184",
+ "pos_x": 7.5625,
+ "pos_y": 13.34375,
+ "pos_z": 55.125,
+ "stations": "Lundwall Silo,Mayr Hangar,Winne Plant"
+ },
+ {
+ "name": "LP 863-19",
+ "pos_x": 4.75,
+ "pos_y": 14.0625,
+ "pos_z": 85.53125,
+ "stations": "Avicenna Terminal,Thornton Terminal,Wallace Vision,Volk Station,Burnet Station,McBride Hub,Willis Colony,Belyayev Hub,Dobzhansky Terminal,Ockels Holdings,Rond d'Alembert Installation,Fullerton Settlement,Bramah's Folly"
+ },
+ {
+ "name": "LP 864-1",
+ "pos_x": -1.09375,
+ "pos_y": 5.84375,
+ "pos_z": 61.21875,
+ "stations": "Bridger Plant,Brillant's Inheritance"
+ },
+ {
+ "name": "LP 869-42",
+ "pos_x": -16.28125,
+ "pos_y": -28.84375,
+ "pos_z": 68.09375,
+ "stations": "Bishop Terminal,Coulomb Orbital"
+ },
+ {
+ "name": "LP 872-8",
+ "pos_x": -34.15625,
+ "pos_y": -62.15625,
+ "pos_z": 80.21875,
+ "stations": "Foucault Ring,Napier Hub,Schmidt Keep,Ricardo Dock,VanderMeer Gateway"
+ },
+ {
+ "name": "LP 876-26",
+ "pos_x": -16.03125,
+ "pos_y": -56.875,
+ "pos_z": 30.6875,
+ "stations": "Galiano Beacon,Haipeng Terminal,White Mines"
+ },
+ {
+ "name": "LP 876-76",
+ "pos_x": -29.53125,
+ "pos_y": -79.375,
+ "pos_z": 38.9375,
+ "stations": "Brunel Ring,McClintock Prospect"
+ },
+ {
+ "name": "LP 878-87",
+ "pos_x": -13.40625,
+ "pos_y": -49.90625,
+ "pos_z": 11.1875,
+ "stations": "Romanenko Refinery,Cassidy Refinery,Behnken Port,Lopez-Alegria City"
+ },
+ {
+ "name": "LP 887-68",
+ "pos_x": 35.03125,
+ "pos_y": -80.75,
+ "pos_z": -38.5,
+ "stations": "Hope Platform,Harvey Outpost"
+ },
+ {
+ "name": "LP 888-62",
+ "pos_x": 49.5,
+ "pos_y": -95.6875,
+ "pos_z": -44.65625,
+ "stations": "Matthaus Olbers Dock,Goodricke Port,Arthur Horizons,Hume Installation"
+ },
+ {
+ "name": "LP 90-39",
+ "pos_x": -18.25,
+ "pos_y": 33.09375,
+ "pos_z": -39.09375,
+ "stations": "Guerrero Terminal,Coulomb Prospect,Robson Terminal,Hermite Hangar"
+ },
+ {
+ "name": "LP 903-21",
+ "pos_x": 70.46875,
+ "pos_y": 25.15625,
+ "pos_z": -4.5,
+ "stations": "Prunariu Installation,Avdeyev Settlement,Liska Depot"
+ },
+ {
+ "name": "LP 906-9",
+ "pos_x": 43.4375,
+ "pos_y": 25.78125,
+ "pos_z": 6.5625,
+ "stations": "Hertz Orbital,Borel Depot,Walker Base,Hawking Enterprise,al-Din Enterprise"
+ },
+ {
+ "name": "LP 907-37",
+ "pos_x": 23.1875,
+ "pos_y": 52.53125,
+ "pos_z": 36.3125,
+ "stations": "Bartlett Plant"
+ },
+ {
+ "name": "LP 908-11",
+ "pos_x": 42.84375,
+ "pos_y": 25.34375,
+ "pos_z": 16.9375,
+ "stations": "So-yeon City,Smith Hub,Caidin Beacon,Huygens Ring"
+ },
+ {
+ "name": "LP 91-140",
+ "pos_x": 38.71875,
+ "pos_y": -35.53125,
+ "pos_z": 9.84375,
+ "stations": "Palmer Refinery,Parise Hub,von Helmont Beacon"
+ },
+ {
+ "name": "LP 917-1",
+ "pos_x": 10.46875,
+ "pos_y": 14.5625,
+ "pos_z": 49.4375,
+ "stations": "Daimler Station,Ferguson Ring,Acharya Landing,Thirsk Port,Kagan Enterprise"
+ },
+ {
+ "name": "LP 917-19",
+ "pos_x": 20.5625,
+ "pos_y": 17.8125,
+ "pos_z": 69.53125,
+ "stations": "Wolfe Terminal,Bresnik Terminal,Eyharts Point"
+ },
+ {
+ "name": "LP 918-29",
+ "pos_x": 17.40625,
+ "pos_y": 12.5625,
+ "pos_z": 89.8125,
+ "stations": "Sakers Point,Smith Gateway,Khan Survey,Novitski Ring"
+ },
+ {
+ "name": "LP 926-40",
+ "pos_x": -9.78125,
+ "pos_y": -26.71875,
+ "pos_z": 45.1875,
+ "stations": "Debye Laboratory,Lee City,Cogswell Enterprise,Cousin Keep,Fernandez Gateway"
+ },
+ {
+ "name": "LP 931-40",
+ "pos_x": -14.84375,
+ "pos_y": -60.4375,
+ "pos_z": 37.1875,
+ "stations": "Lerner Terminal,Fernandes Vision,Norman Enterprise,Elcano Horizons,Frimout Relay"
+ },
+ {
+ "name": "LP 932-12",
+ "pos_x": -7.9375,
+ "pos_y": -40.09375,
+ "pos_z": 21,
+ "stations": "Blackwell Horizons,Hurston Hub"
+ },
+ {
+ "name": "LP 933-24",
+ "pos_x": -14.71875,
+ "pos_y": -95.59375,
+ "pos_z": 41.875,
+ "stations": "Alfven Platform,Vancouver's Claim,McNaught Dock,Hutchinson Penal colony"
+ },
+ {
+ "name": "LP 937-31",
+ "pos_x": 8.71875,
+ "pos_y": -188.46875,
+ "pos_z": 24.8125,
+ "stations": "Dawes Dock"
+ },
+ {
+ "name": "LP 940-115",
+ "pos_x": 35.21875,
+ "pos_y": -120.09375,
+ "pos_z": -8.78125,
+ "stations": "May City,Hillary Silo,Theodor Winnecke Station,Giles Settlement"
+ },
+ {
+ "name": "LP 941-16",
+ "pos_x": 27.9375,
+ "pos_y": -89.0625,
+ "pos_z": -15.09375,
+ "stations": "King Ring,Walker Terminal,Poincare Arsenal,Peral Prospect,Bryusov Enterprise"
+ },
+ {
+ "name": "LP 943-72",
+ "pos_x": 30.375,
+ "pos_y": -55.28125,
+ "pos_z": -18.6875,
+ "stations": "Sheremetevsky Installation,Lawhead Silo,Reinhold Hub"
+ },
+ {
+ "name": "LP 98-132",
+ "pos_x": -26.78125,
+ "pos_y": 37.03125,
+ "pos_z": -4.59375,
+ "stations": "Freeport,Prospect Five"
+ },
+ {
+ "name": "LP 98-62",
+ "pos_x": 94.09375,
+ "pos_y": -27.5625,
+ "pos_z": 22.21875,
+ "stations": "Covey Horizons,Howe Orbital,Ordway Silo"
+ },
+ {
+ "name": "LP Draconis",
+ "pos_x": -87.9375,
+ "pos_y": 49.34375,
+ "pos_z": -15.5,
+ "stations": "Dobrovolski Enterprise,Carter's Inheritance"
+ },
+ {
+ "name": "LPM 229",
+ "pos_x": 78.34375,
+ "pos_y": -42.96875,
+ "pos_z": -2.21875,
+ "stations": "Maler Lab,Kidinnu Hub,Fehrenbach Refinery,Macan Reach"
+ },
+ {
+ "name": "LPM 26",
+ "pos_x": 38.40625,
+ "pos_y": -66.0625,
+ "pos_z": 28.15625,
+ "stations": "Bell Landing,Hirn Prospect,Rand Base"
+ },
+ {
+ "name": "LPM 561",
+ "pos_x": 17.5625,
+ "pos_y": 42.625,
+ "pos_z": 67.5625,
+ "stations": "Hermaszewski Settlement,Hodgson Oasis"
+ },
+ {
+ "name": "LPM 607",
+ "pos_x": 23.1875,
+ "pos_y": 8,
+ "pos_z": 76.46875,
+ "stations": "Block Orbital,Atwood Settlement"
+ },
+ {
+ "name": "LPM 696",
+ "pos_x": 20.5625,
+ "pos_y": -30.625,
+ "pos_z": 60.5625,
+ "stations": "Peterson Refinery,Dalmas Beacon,Remek Colony"
+ },
+ {
+ "name": "LPM 746",
+ "pos_x": -25.65625,
+ "pos_y": -43.71875,
+ "pos_z": 58.90625,
+ "stations": "Merbold Port,Wood Lab,Zudov City"
+ },
+ {
+ "name": "LPM 752",
+ "pos_x": 28.125,
+ "pos_y": -57.25,
+ "pos_z": 64.21875,
+ "stations": "Eisinga Horizons,Bond Colony"
+ },
+ {
+ "name": "LQ Hydrae",
+ "pos_x": 48.34375,
+ "pos_y": 29.15625,
+ "pos_z": -22.34375,
+ "stations": "Wohler Horizons"
+ },
+ {
+ "name": "LR Hydrae",
+ "pos_x": 85.0625,
+ "pos_y": 70.53125,
+ "pos_z": -16.1875,
+ "stations": "Dickson Dock,Wells Point,Caselberg Arena"
+ },
+ {
+ "name": "LS 521",
+ "pos_x": 2.375,
+ "pos_y": 26.5625,
+ "pos_z": -58.6875,
+ "stations": "Ramon Dock"
+ },
+ {
+ "name": "LSE 239",
+ "pos_x": 22.8125,
+ "pos_y": -22.5625,
+ "pos_z": 46.15625,
+ "stations": "Eddington Refinery,Makarov Station,Menzies' Inheritance"
+ },
+ {
+ "name": "LTT 1015",
+ "pos_x": -0.84375,
+ "pos_y": -110.46875,
+ "pos_z": -38.3125,
+ "stations": "Fischer Point"
+ },
+ {
+ "name": "LTT 10410",
+ "pos_x": -64.84375,
+ "pos_y": -70.875,
+ "pos_z": -52.25,
+ "stations": "Reed Survey,Ellern Point"
+ },
+ {
+ "name": "LTT 1044",
+ "pos_x": 39.84375,
+ "pos_y": -77.75,
+ "pos_z": 6.96875,
+ "stations": "Grego Plant,Ryle Prospect"
+ },
+ {
+ "name": "LTT 10462",
+ "pos_x": -46.71875,
+ "pos_y": -46.25,
+ "pos_z": -40.34375,
+ "stations": "Belyanin Station,Giles Station"
+ },
+ {
+ "name": "LTT 10482",
+ "pos_x": -61.1875,
+ "pos_y": 23.59375,
+ "pos_z": -42,
+ "stations": "Rotsler Landing"
+ },
+ {
+ "name": "LTT 10823",
+ "pos_x": -24.5,
+ "pos_y": -81.15625,
+ "pos_z": -70.09375,
+ "stations": "Faris Landing,Ray Hub"
+ },
+ {
+ "name": "LTT 10918",
+ "pos_x": -60.53125,
+ "pos_y": -9.0625,
+ "pos_z": -73.78125,
+ "stations": "Fujimori City,Fuca Relay"
+ },
+ {
+ "name": "LTT 11103",
+ "pos_x": -5.125,
+ "pos_y": -71.28125,
+ "pos_z": -30.46875,
+ "stations": "Korniyenko Terminal"
+ },
+ {
+ "name": "LTT 11244",
+ "pos_x": -4.1875,
+ "pos_y": -36.65625,
+ "pos_z": -57.09375,
+ "stations": "Delany City,Leiber Installation,Stasheff City,Morgan Hub,Russell Hub,Offutt Station,Williams Enterprise,Watts Settlement,Carver Prospect,Bryant Holdings,Moffitt Dock,Jones Orbital,Russell Relay,Kingsmill Terminal,Crichton Dock"
+ },
+ {
+ "name": "LTT 11383",
+ "pos_x": -25.1875,
+ "pos_y": -14.5,
+ "pos_z": -81.84375,
+ "stations": "Nelson Platform,Morgan Terminal,Curie Refinery"
+ },
+ {
+ "name": "LTT 11455",
+ "pos_x": -17.84375,
+ "pos_y": -11.46875,
+ "pos_z": -72.84375,
+ "stations": "Ising Depot,Stebler Mines,Goeschke Station"
+ },
+ {
+ "name": "LTT 1147",
+ "pos_x": -8.46875,
+ "pos_y": -63.34375,
+ "pos_z": -37.53125,
+ "stations": "Gordon Terminal,Murray Base,Bramah Base"
+ },
+ {
+ "name": "LTT 11478",
+ "pos_x": 12.1875,
+ "pos_y": -38.8125,
+ "pos_z": -91.125,
+ "stations": "Heng Port,Aristotle Port,Chaudhary Enterprise,Zholobov Enterprise,Kuipers Terminal,White Terminal,Ross Hub,Hernandez Port,Kekule Enterprise,Piserchia Colony,Rodrigues Town,Barba Hub,Mastracchio Terminal"
+ },
+ {
+ "name": "LTT 11485",
+ "pos_x": -26.78125,
+ "pos_y": -8.53125,
+ "pos_z": -100.09375,
+ "stations": "Nespoli Ring,Bondar Hub,Acton Terminal,Nasmyth Dock"
+ },
+ {
+ "name": "LTT 11503",
+ "pos_x": -10.5,
+ "pos_y": -13,
+ "pos_z": -70.5625,
+ "stations": "Fung Outpost"
+ },
+ {
+ "name": "LTT 11519",
+ "pos_x": -6.65625,
+ "pos_y": -16.78125,
+ "pos_z": -81.6875,
+ "stations": "Edison Beacon,Jendrassik Dock"
+ },
+ {
+ "name": "LTT 11571",
+ "pos_x": 11.96875,
+ "pos_y": -26.9375,
+ "pos_z": -93.34375,
+ "stations": "Scully-Power Terminal,Drebbel Station"
+ },
+ {
+ "name": "LTT 11648",
+ "pos_x": -11.0625,
+ "pos_y": 0.03125,
+ "pos_z": -109.875,
+ "stations": "Feustel Orbital,Wiberg Hub,Savinykh Horizons,Linge Relay,Howard Horizons"
+ },
+ {
+ "name": "LTT 11655",
+ "pos_x": 19.6875,
+ "pos_y": -18.59375,
+ "pos_z": -90.4375,
+ "stations": "Hodgkin Orbital,Marshall Dock,Knipling Ring"
+ },
+ {
+ "name": "LTT 1190",
+ "pos_x": 29.5625,
+ "pos_y": -76.5,
+ "pos_z": -10.65625,
+ "stations": "Bosch Dock,Marconi's Folly,Bloomfield Refinery"
+ },
+ {
+ "name": "LTT 11926",
+ "pos_x": -35.15625,
+ "pos_y": 43.34375,
+ "pos_z": -98.5625,
+ "stations": "Forward Mines,Slonczewski Enterprise"
+ },
+ {
+ "name": "LTT 11958",
+ "pos_x": -64.5625,
+ "pos_y": 51.625,
+ "pos_z": -76,
+ "stations": "Pierres' Pride,Sagan Point,Macdonald Port"
+ },
+ {
+ "name": "LTT 11993",
+ "pos_x": -38.9375,
+ "pos_y": 50.0625,
+ "pos_z": -94.03125,
+ "stations": "Tennyson d'Eyncourt Depot,Solovyov Station"
+ },
+ {
+ "name": "LTT 12033",
+ "pos_x": 27.03125,
+ "pos_y": 18.53125,
+ "pos_z": -59.4375,
+ "stations": "Thorne Port,Walheim Plant"
+ },
+ {
+ "name": "LTT 12058",
+ "pos_x": 37.09375,
+ "pos_y": 15.875,
+ "pos_z": -54.6875,
+ "stations": "Yurchikhin Orbital,Shatalov Market,Solovyov Port,Bean Enterprise,Nicollier Station,Levchenko Port,Parise Station,Haber Station,Bethe Station"
+ },
+ {
+ "name": "LTT 12294",
+ "pos_x": 71.125,
+ "pos_y": 54.75,
+ "pos_z": -72.8125,
+ "stations": "Haignere Ring,Boe Port,Cole Barracks"
+ },
+ {
+ "name": "LTT 12360",
+ "pos_x": 46.03125,
+ "pos_y": 40.03125,
+ "pos_z": -48.03125,
+ "stations": "Bordage Landing,Benyovszky Terminal,Burgess Vision,Miletus Keep"
+ },
+ {
+ "name": "LTT 12378",
+ "pos_x": -13.4375,
+ "pos_y": 78.0625,
+ "pos_z": -84.71875,
+ "stations": "Levi-Strauss Terminal,Sinclair Relay,Laval Survey,Clement Prospect"
+ },
+ {
+ "name": "LTT 12449",
+ "pos_x": 16.75,
+ "pos_y": 44.46875,
+ "pos_z": -44.6875,
+ "stations": "Rennie Prospect"
+ },
+ {
+ "name": "LTT 12680",
+ "pos_x": 31.09375,
+ "pos_y": 53.25,
+ "pos_z": -34.96875,
+ "stations": "Weber Enterprise"
+ },
+ {
+ "name": "LTT 12696",
+ "pos_x": 23.6875,
+ "pos_y": 77,
+ "pos_z": -51.375,
+ "stations": "Low Orbital,Hill Camp,Dashiell Installation"
+ },
+ {
+ "name": "LTT 12700",
+ "pos_x": -20.25,
+ "pos_y": 82.4375,
+ "pos_z": -63.53125,
+ "stations": "Janifer Hub,Alexandrov Ring,Martinez Gateway,Bernoulli Observatory,Hillary Relay"
+ },
+ {
+ "name": "LTT 1272",
+ "pos_x": 26.125,
+ "pos_y": -95.15625,
+ "pos_z": -32.65625,
+ "stations": "Murakami Ring"
+ },
+ {
+ "name": "LTT 12723",
+ "pos_x": 69.96875,
+ "pos_y": 79.71875,
+ "pos_z": -41.65625,
+ "stations": "Temple Bastion,Buckell Hub,al-Din Enterprise"
+ },
+ {
+ "name": "LTT 12734",
+ "pos_x": 7.15625,
+ "pos_y": 63.6875,
+ "pos_z": -43.8125,
+ "stations": "Dedekind Vision,Kuttner Landing,Jeschke Hub"
+ },
+ {
+ "name": "LTT 12787",
+ "pos_x": 23.25,
+ "pos_y": 86.3125,
+ "pos_z": -47.59375,
+ "stations": "Bailey Dock,Eisenstein Landing,Quimby Relay"
+ },
+ {
+ "name": "LTT 12874",
+ "pos_x": 62.59375,
+ "pos_y": 91.40625,
+ "pos_z": -28.59375,
+ "stations": "Battuta Colony"
+ },
+ {
+ "name": "LTT 1289",
+ "pos_x": 120.40625,
+ "pos_y": -115.21875,
+ "pos_z": 52.40625,
+ "stations": "Wallis Hub,Barnes Installation,Fraunhofer Station,Dunlop Vision,Reber Hub,Roskam Orbital,Pannekoek Port,Osterbrock Terminal,Michell Ring,Sato's Progress,Hirayama Vision"
+ },
+ {
+ "name": "LTT 12925",
+ "pos_x": 14.53125,
+ "pos_y": 81.78125,
+ "pos_z": -34.59375,
+ "stations": "Braun Dock,Fuglesang Landing"
+ },
+ {
+ "name": "LTT 12990",
+ "pos_x": -0.5625,
+ "pos_y": 67.40625,
+ "pos_z": -29.65625,
+ "stations": "Sylvester Dock,Ostrander Hub"
+ },
+ {
+ "name": "LTT 13125",
+ "pos_x": -43.09375,
+ "pos_y": 86.875,
+ "pos_z": -48.90625,
+ "stations": "Hamilton Hub,Ross Port,Markham Orbital,McHugh Depot,Grimwood Beacon,Dowling Port,Charnas Terminal,Morgan Terminal,Alvarez de Pineda Orbital,Springer City,Griffin Point"
+ },
+ {
+ "name": "LTT 13232",
+ "pos_x": 46.625,
+ "pos_y": 84.65625,
+ "pos_z": -1.125,
+ "stations": "Whitney Platform,Anderson Platform"
+ },
+ {
+ "name": "LTT 13380",
+ "pos_x": 45.46875,
+ "pos_y": 99.4375,
+ "pos_z": 7.5625,
+ "stations": "Morin Station,Chamitoff Hub,Dias' Inheritance,Grabe Dock"
+ },
+ {
+ "name": "LTT 13415",
+ "pos_x": 36.375,
+ "pos_y": 86.9375,
+ "pos_z": 7.5,
+ "stations": "Cartwright Station,Evans Terminal,Nachtigal Survey,Lounge Keep"
+ },
+ {
+ "name": "LTT 1345",
+ "pos_x": 92.625,
+ "pos_y": -124.1875,
+ "pos_z": 12.90625,
+ "stations": "Phaid,Rankin Refinery"
+ },
+ {
+ "name": "LTT 1349",
+ "pos_x": 17.15625,
+ "pos_y": -66.96875,
+ "pos_z": -29.3125,
+ "stations": "Lundwall City,Greenstein Bastion,Gelfand Survey,Aitken Vision,Reed Terminal"
+ },
+ {
+ "name": "LTT 135",
+ "pos_x": 22.03125,
+ "pos_y": -113,
+ "pos_z": 28.375,
+ "stations": "Princess Palace,Agnew's Folly,Kagawa Prospect,Farghani Base,Mattei Holdings"
+ },
+ {
+ "name": "LTT 1353",
+ "pos_x": 8.5625,
+ "pos_y": -81.96875,
+ "pos_z": -50.46875,
+ "stations": "Williamson Terminal,Judson Installation,Hillary Enterprise"
+ },
+ {
+ "name": "LTT 1373",
+ "pos_x": 78.625,
+ "pos_y": -122.59375,
+ "pos_z": -5.5,
+ "stations": "Wilson Dock"
+ },
+ {
+ "name": "LTT 13866",
+ "pos_x": 30.6875,
+ "pos_y": 101.40625,
+ "pos_z": 37.46875,
+ "stations": "Lebedev Hub"
+ },
+ {
+ "name": "LTT 13904",
+ "pos_x": -23.4375,
+ "pos_y": 102.65625,
+ "pos_z": 0.53125,
+ "stations": "McDonald Dock,Friend Gateway,Hendel Port,Wagner Vision,Killough Beacon,Brooks Installation,Burckhardt Installation"
+ },
+ {
+ "name": "LTT 1391",
+ "pos_x": 7.0625,
+ "pos_y": -96.03125,
+ "pos_z": -68.34375,
+ "stations": "Hiroyuki's Claim,Roggeveen Vision"
+ },
+ {
+ "name": "LTT 14019",
+ "pos_x": -38.375,
+ "pos_y": 66.25,
+ "pos_z": -12.96875,
+ "stations": "Kiernan Barracks,Ballard Gateway,Wilder Survey,Rond d'Alembert Observatory,Robinson Installation"
+ },
+ {
+ "name": "LTT 14094",
+ "pos_x": -35.46875,
+ "pos_y": 85.71875,
+ "pos_z": 0.9375,
+ "stations": "Skolem Colony,Guerrero Installation,Cavendish Refinery,Asimov Settlement"
+ },
+ {
+ "name": "LTT 141",
+ "pos_x": 50.28125,
+ "pos_y": 93.0625,
+ "pos_z": 38.9375,
+ "stations": "Butz Terminal"
+ },
+ {
+ "name": "LTT 14174",
+ "pos_x": -5.78125,
+ "pos_y": 103.65625,
+ "pos_z": 43.34375,
+ "stations": "Kavandi Orbital,Ejeta Enterprise,Birdseye Plant,Vaucanson Hub,Piccard Landing"
+ },
+ {
+ "name": "LTT 14271",
+ "pos_x": -31.625,
+ "pos_y": 70.125,
+ "pos_z": 8.71875,
+ "stations": "Hutchinson Landing,Clarke Bastion,North Landing,Panshin Beacon"
+ },
+ {
+ "name": "LTT 14398",
+ "pos_x": -5.03125,
+ "pos_y": 63.5,
+ "pos_z": 41.125,
+ "stations": "Komarov Terminal,Vian Depot,Harness Base,Jordan Base"
+ },
+ {
+ "name": "LTT 14429",
+ "pos_x": -5.96875,
+ "pos_y": 91.3125,
+ "pos_z": 66.375,
+ "stations": "Winne Refinery,Marshall Survey,Chilton Dock"
+ },
+ {
+ "name": "LTT 14436",
+ "pos_x": -8.09375,
+ "pos_y": 90.75,
+ "pos_z": 64.34375,
+ "stations": "Egan Plant,Galiano City"
+ },
+ {
+ "name": "LTT 14437",
+ "pos_x": -44.3125,
+ "pos_y": 68.6875,
+ "pos_z": 4.59375,
+ "stations": "Bridger Base"
+ },
+ {
+ "name": "LTT 14474",
+ "pos_x": -68,
+ "pos_y": 60.46875,
+ "pos_z": -28.40625,
+ "stations": "Piccard Hub"
+ },
+ {
+ "name": "LTT 14478",
+ "pos_x": -25.09375,
+ "pos_y": 62.8125,
+ "pos_z": 24.65625,
+ "stations": "Resnick Market,Stein Enterprise,Godwin Point,Lanier City,McAllaster Port"
+ },
+ {
+ "name": "LTT 14493",
+ "pos_x": -51.0625,
+ "pos_y": 95.34375,
+ "pos_z": 24.9375,
+ "stations": "Lukyanenko Plant,Sargent Depot,Savorgnan de Brazza Landing"
+ },
+ {
+ "name": "LTT 1483",
+ "pos_x": 31.09375,
+ "pos_y": -88.53125,
+ "pos_z": -44.3125,
+ "stations": "Hedin Gateway,Cerulli Bastion"
+ },
+ {
+ "name": "LTT 14850",
+ "pos_x": -24,
+ "pos_y": 51.3125,
+ "pos_z": 63.28125,
+ "stations": "Franklin Vision,Runco Station,Howard Keep,Allen Vista"
+ },
+ {
+ "name": "LTT 149",
+ "pos_x": -26.21875,
+ "pos_y": -70.96875,
+ "pos_z": -5.21875,
+ "stations": "Hiraga Hub,Bao Arsenal"
+ },
+ {
+ "name": "LTT 14971",
+ "pos_x": -77.125,
+ "pos_y": 66.5,
+ "pos_z": 17.5,
+ "stations": "Christian Colony,Tilley's Claim,Goulart Base"
+ },
+ {
+ "name": "LTT 14976",
+ "pos_x": -70.53125,
+ "pos_y": 66.59375,
+ "pos_z": 40.90625,
+ "stations": "Andrews Dock,Bergerac Depot"
+ },
+ {
+ "name": "LTT 15068",
+ "pos_x": -34.03125,
+ "pos_y": 38,
+ "pos_z": 82.5,
+ "stations": "Kennan Enterprise,Grabe Station,Reiter Hub"
+ },
+ {
+ "name": "LTT 15084",
+ "pos_x": -72.5625,
+ "pos_y": 62.3125,
+ "pos_z": 69.46875,
+ "stations": "Hermite Depot"
+ },
+ {
+ "name": "LTT 1509",
+ "pos_x": 53.875,
+ "pos_y": -82.625,
+ "pos_z": -12.4375,
+ "stations": "Payne-Scott Enterprise,Christian Prospect,Arp Dock,Janifer Landing"
+ },
+ {
+ "name": "LTT 15225",
+ "pos_x": -71.375,
+ "pos_y": 46.5,
+ "pos_z": 55.65625,
+ "stations": "Mitchell Terminal,Wedge Barracks,Garcia Hub,Bunch Gateway,Hiraga Port,Cortes Orbital,Smeaton Observatory,Kornbluth Installation"
+ },
+ {
+ "name": "LTT 15278",
+ "pos_x": -63.71875,
+ "pos_y": 35.8125,
+ "pos_z": 85.0625,
+ "stations": "Zholobov Enterprise,Mitchell Installation,Alvarez de Pineda Enterprise"
+ },
+ {
+ "name": "LTT 15294",
+ "pos_x": -84.6875,
+ "pos_y": 50.21875,
+ "pos_z": -6.40625,
+ "stations": "Huygens Dock,Papin Refinery,Salam Settlement"
+ },
+ {
+ "name": "LTT 15449",
+ "pos_x": -55.28125,
+ "pos_y": 20.5,
+ "pos_z": 42.15625,
+ "stations": "Roentgen Port,Binet Port,Malerba Station,Dashiell Depot,Barry Terminal,Faraday Dock,Hackworth Hub,Bulgarin Landing,Guidoni Orbital,Reilly Dock,Detmer Landing,Bose Station"
+ },
+ {
+ "name": "LTT 15461",
+ "pos_x": -55.9375,
+ "pos_y": 13.21875,
+ "pos_z": 69.375,
+ "stations": "Thomson Port,O'Connor Terminal,Qurra Hub,Stephenson Installation,Reed Point"
+ },
+ {
+ "name": "LTT 15574",
+ "pos_x": -55.9375,
+ "pos_y": 1.9375,
+ "pos_z": 63.59375,
+ "stations": "Haxel Port,Marconi Survey,Yaping Dock,Descartes Gateway,Cormack Enterprise"
+ },
+ {
+ "name": "LTT 15587",
+ "pos_x": -78.53125,
+ "pos_y": 0.9375,
+ "pos_z": 87.53125,
+ "stations": "Bramah Gateway,Kidman Prospect,Bouch Ring,Gresley Port,Yamazaki City,Comino Orbital,Kuipers Penal colony,Campbell Observatory,Buckland Dock"
+ },
+ {
+ "name": "LTT 15693",
+ "pos_x": -104.75,
+ "pos_y": 12.5625,
+ "pos_z": 47.71875,
+ "stations": "Vonarburg Mines,May Enterprise,VanderMeer Stop"
+ },
+ {
+ "name": "LTT 1572",
+ "pos_x": 81.9375,
+ "pos_y": -112.5625,
+ "pos_z": -11.34375,
+ "stations": "Young Dock,Tall Refinery,Corben Relay"
+ },
+ {
+ "name": "LTT 1581",
+ "pos_x": 44.21875,
+ "pos_y": -96.5625,
+ "pos_z": -45.125,
+ "stations": "Keyes Retreat,Gwynn Stop"
+ },
+ {
+ "name": "LTT 1582",
+ "pos_x": 44.5,
+ "pos_y": -96.96875,
+ "pos_z": -45.21875,
+ "stations": "Pimi Ring"
+ },
+ {
+ "name": "LTT 15838",
+ "pos_x": -80.21875,
+ "pos_y": 0.25,
+ "pos_z": 34.09375,
+ "stations": "Coleman Terminal"
+ },
+ {
+ "name": "LTT 15899",
+ "pos_x": -60.34375,
+ "pos_y": -20.46875,
+ "pos_z": 53.40625,
+ "stations": "Schweickart Enterprise,Onufriyenko Station,Kekule Orbital"
+ },
+ {
+ "name": "LTT 1598",
+ "pos_x": 38.53125,
+ "pos_y": -79.1875,
+ "pos_z": -35.84375,
+ "stations": "Laumer Forum,Qushji Gateway,Borrego City,Starzl Legacy,Cowling Works,Watson Dock,Covington Dock,Ernst Terminal,Nesvadba Settlement"
+ },
+ {
+ "name": "LTT 15985",
+ "pos_x": -71.6875,
+ "pos_y": -10.15625,
+ "pos_z": 28.15625,
+ "stations": "Akiyama Hangar,Creighton Vision"
+ },
+ {
+ "name": "LTT 16016",
+ "pos_x": -62.53125,
+ "pos_y": 12.375,
+ "pos_z": -5.5,
+ "stations": "Smith Colony,Lee Station,Capek Horizons,Gelfand Barracks"
+ },
+ {
+ "name": "LTT 16019",
+ "pos_x": -67.4375,
+ "pos_y": -15,
+ "pos_z": 31.34375,
+ "stations": "Wilson Installation,Hartog Relay,Rey Prospect"
+ },
+ {
+ "name": "LTT 16184",
+ "pos_x": -101.28125,
+ "pos_y": -22.46875,
+ "pos_z": 25.03125,
+ "stations": "Minkowski City,Hartog Vision"
+ },
+ {
+ "name": "LTT 16218",
+ "pos_x": -59.28125,
+ "pos_y": -35.5625,
+ "pos_z": 37,
+ "stations": "Chargaff Port,Tuan's Inheritance"
+ },
+ {
+ "name": "LTT 16301",
+ "pos_x": -93,
+ "pos_y": -13.1875,
+ "pos_z": 3.5625,
+ "stations": "Ostrander Landing,Cobb Vision,Maury Port"
+ },
+ {
+ "name": "LTT 16385",
+ "pos_x": -67.9375,
+ "pos_y": -61.9375,
+ "pos_z": 38.78125,
+ "stations": "Morgan Port"
+ },
+ {
+ "name": "LTT 16422",
+ "pos_x": -82.09375,
+ "pos_y": -74.4375,
+ "pos_z": 41.875,
+ "stations": "Borlaug Station,Huygens Dock,Yu Point"
+ },
+ {
+ "name": "LTT 16456",
+ "pos_x": -56.59375,
+ "pos_y": -49.5,
+ "pos_z": 26,
+ "stations": "Ray Platform,Morris Reach,Cabrera Port"
+ },
+ {
+ "name": "LTT 1652",
+ "pos_x": 14.75,
+ "pos_y": -54.65625,
+ "pos_z": -44.59375,
+ "stations": "Benz Keep,Wheelock Holdings,Jensen Escape,Haberlandt City"
+ },
+ {
+ "name": "LTT 16522",
+ "pos_x": -51.125,
+ "pos_y": -34.625,
+ "pos_z": 11.875,
+ "stations": "Bluford Dock,Underwood Relay"
+ },
+ {
+ "name": "LTT 16523",
+ "pos_x": -72.65625,
+ "pos_y": 13.28125,
+ "pos_z": -25.9375,
+ "stations": "Ivins Market,Arrhenius Orbital,Bohm Hub,Curbeam Terminal,Roberts Terminal,Kondakova Ring,Citi Legacy,Hale Vision,Tepper Works,Jacquard Orbital,Kraepelin Gateway,Stott Dock,Pike Gateway"
+ },
+ {
+ "name": "LTT 16548",
+ "pos_x": -81.125,
+ "pos_y": -68.21875,
+ "pos_z": 23.125,
+ "stations": "Tyson Gateway,Cooper Installation"
+ },
+ {
+ "name": "LTT 16741",
+ "pos_x": -88.375,
+ "pos_y": -67.8125,
+ "pos_z": 2.40625,
+ "stations": "Yegorov City,Gibson's Inheritance"
+ },
+ {
+ "name": "LTT 16764",
+ "pos_x": -89.5,
+ "pos_y": -2.46875,
+ "pos_z": -31.40625,
+ "stations": "Virtanen Ring,Smith Orbital"
+ },
+ {
+ "name": "LTT 1678",
+ "pos_x": 19.96875,
+ "pos_y": -28.03125,
+ "pos_z": -6.5,
+ "stations": "Vishweswarayya Station,Comino Enterprise,Emshwiller Vision,Taylor Orbital,Barth Penal colony"
+ },
+ {
+ "name": "LTT 16910",
+ "pos_x": -72.375,
+ "pos_y": -39.65625,
+ "pos_z": -18.125,
+ "stations": "Cayley Gateway,Mendez Ring"
+ },
+ {
+ "name": "LTT 16979",
+ "pos_x": -97.25,
+ "pos_y": 24.3125,
+ "pos_z": -52.5625,
+ "stations": "Adamson Platform,Bohm Settlement"
+ },
+ {
+ "name": "LTT 17102",
+ "pos_x": -73.84375,
+ "pos_y": 16.5625,
+ "pos_z": -45.59375,
+ "stations": "Velazquez Ring,Gamow Settlement"
+ },
+ {
+ "name": "LTT 17149",
+ "pos_x": -51.59375,
+ "pos_y": -48.5,
+ "pos_z": -30.59375,
+ "stations": "White Terminal,England Base,Isherwood Mines"
+ },
+ {
+ "name": "LTT 17156",
+ "pos_x": -52.03125,
+ "pos_y": -44.4375,
+ "pos_z": -32.78125,
+ "stations": "Timofeyevich Ring,Ledyard Ring,Kimberlin Orbital,Euclid's Progress,Joule Beacon"
+ },
+ {
+ "name": "LTT 1739",
+ "pos_x": 71.34375,
+ "pos_y": -84.09375,
+ "pos_z": -7.5625,
+ "stations": "Clarke Port,Shosuke Camp,Gamow Base,Diophantus Dock,Zillig Gateway"
+ },
+ {
+ "name": "LTT 17422",
+ "pos_x": -17.25,
+ "pos_y": -60.15625,
+ "pos_z": -65.25,
+ "stations": "Low Enterprise,Rosenberg Hub,Seega Port,Hopkinson Survey,Khayyam Dock"
+ },
+ {
+ "name": "LTT 17686",
+ "pos_x": 14.75,
+ "pos_y": -27.1875,
+ "pos_z": -53.21875,
+ "stations": "Antonelli Lab,Bresnik Station,Tsibliyev Landing,Herbert Survey"
+ },
+ {
+ "name": "LTT 17817",
+ "pos_x": 95.125,
+ "pos_y": -61.34375,
+ "pos_z": 29.5625,
+ "stations": "Searle Dock,Bolton Hub,Jones City,Penzias Point,Dowty Terminal,Jones Gateway,Antonio de Andrade Exchange,Hynek Hub,Pearse Terminal,Espenak Orbital,Rutan Vision,Yuzhe Hub,Williams Colony"
+ },
+ {
+ "name": "LTT 17868",
+ "pos_x": 22.78125,
+ "pos_y": -12.90625,
+ "pos_z": -67.59375,
+ "stations": "Giles Colony,Pierce Hangar,Temple Penal colony"
+ },
+ {
+ "name": "LTT 1798",
+ "pos_x": 39.1875,
+ "pos_y": -72.9375,
+ "pos_z": -49.28125,
+ "stations": "Gilliland Ring,Yourkevitch Dock,Cramer Terminal"
+ },
+ {
+ "name": "LTT 18",
+ "pos_x": 31.25,
+ "pos_y": -135,
+ "pos_z": 43.0625,
+ "stations": "Hirayama City,Antoniadi Barracks"
+ },
+ {
+ "name": "LTT 18075",
+ "pos_x": -39.25,
+ "pos_y": 64.875,
+ "pos_z": -72.8125,
+ "stations": "Hughes-Fulford Terminal,Wheelock Port,Brunel Ring"
+ },
+ {
+ "name": "LTT 18486",
+ "pos_x": -45.09375,
+ "pos_y": -11.96875,
+ "pos_z": 56.46875,
+ "stations": "Boswell Platform,Macarthur Terminal"
+ },
+ {
+ "name": "LTT 18626",
+ "pos_x": -55.09375,
+ "pos_y": -45.1875,
+ "pos_z": -12.5625,
+ "stations": "Guest Orbital,Block Enterprise,Pohl Lab"
+ },
+ {
+ "name": "LTT 1873",
+ "pos_x": 104.28125,
+ "pos_y": -127.1875,
+ "pos_z": -49.21875,
+ "stations": "Celsius Gateway,Hadid City,Lippisch Hub,Krupkat Holdings,Molchanov Terminal,Garrido Ring,Frobenius Landing,Theodorsen Station,Utkin City,Morgan Base"
+ },
+ {
+ "name": "LTT 1935",
+ "pos_x": 48.75,
+ "pos_y": -50.09375,
+ "pos_z": -11.84375,
+ "stations": "Wolf Port,Mohun Relay"
+ },
+ {
+ "name": "LTT 198",
+ "pos_x": 2.53125,
+ "pos_y": -71.625,
+ "pos_z": 9.71875,
+ "stations": "Keyes Beacon,Dini,Marcusa 242"
+ },
+ {
+ "name": "LTT 2042",
+ "pos_x": 46,
+ "pos_y": -62.78125,
+ "pos_z": -73.65625,
+ "stations": "Vinge Relay,Gaffney Refinery"
+ },
+ {
+ "name": "LTT 2062",
+ "pos_x": 62.84375,
+ "pos_y": -64.125,
+ "pos_z": -42.96875,
+ "stations": "Keeler Vision"
+ },
+ {
+ "name": "LTT 2099",
+ "pos_x": 49.5625,
+ "pos_y": -59.375,
+ "pos_z": -78.59375,
+ "stations": "Grant Enterprise,Fernandez Gateway,Lee Port,Drummond Port,Menzies Terminal,Wachmann Gateway,Hutton Depot"
+ },
+ {
+ "name": "LTT 2151",
+ "pos_x": 15.71875,
+ "pos_y": -15.0625,
+ "pos_z": -17.59375,
+ "stations": "Meucci Port,Veach Point,Read Terminal,Teller Works,Noriega Station,Onnes Depot,Mattingly City,Kessel Works"
+ },
+ {
+ "name": "LTT 2240",
+ "pos_x": 43.375,
+ "pos_y": -35.59375,
+ "pos_z": -55.96875,
+ "stations": "Tyson Market"
+ },
+ {
+ "name": "LTT 2262",
+ "pos_x": 85.03125,
+ "pos_y": -61.28125,
+ "pos_z": -52.8125,
+ "stations": "Bella Camp"
+ },
+ {
+ "name": "LTT 2277",
+ "pos_x": 73.875,
+ "pos_y": -53.46875,
+ "pos_z": -72.65625,
+ "stations": "Stechkin Laboratory,Michalitsianos Platform"
+ },
+ {
+ "name": "LTT 2322",
+ "pos_x": 79.09375,
+ "pos_y": -49.9375,
+ "pos_z": 35.34375,
+ "stations": "Ivins Enterprise,Patsayev Dock,Morey Orbital,Alten Settlement,Hubble Hub,Glazkov Enterprise,Broglie Gateway,Henderson Works,Obruchev Enterprise,Noli Prospect"
+ },
+ {
+ "name": "LTT 2337",
+ "pos_x": 90.4375,
+ "pos_y": -57.625,
+ "pos_z": 3.21875,
+ "stations": "Weaver Laboratory,Bowen Terminal,Alfven Point,Hall Station"
+ },
+ {
+ "name": "LTT 2379",
+ "pos_x": 56.6875,
+ "pos_y": -33.9375,
+ "pos_z": -14.71875,
+ "stations": "Drebbel Terminal,Sharipov Relay"
+ },
+ {
+ "name": "LTT 2396",
+ "pos_x": 33.8125,
+ "pos_y": -17.34375,
+ "pos_z": -54.28125,
+ "stations": "Filter Settlement,Mendeleev Hub,Galton Vision,Akers Colony,Kaleri Platform"
+ },
+ {
+ "name": "LTT 2412",
+ "pos_x": 97.125,
+ "pos_y": -55.6875,
+ "pos_z": -16.5625,
+ "stations": "Velho Installation"
+ },
+ {
+ "name": "LTT 2513",
+ "pos_x": 65.34375,
+ "pos_y": -22.5625,
+ "pos_z": -72.5,
+ "stations": "Hughes-Fulford Ring,Kadenyuk Gateway,MacLean Vision"
+ },
+ {
+ "name": "LTT 2545",
+ "pos_x": 56.28125,
+ "pos_y": -10.90625,
+ "pos_z": -93.3125,
+ "stations": "Stevin's Pride"
+ },
+ {
+ "name": "LTT 2581",
+ "pos_x": 67.59375,
+ "pos_y": -27.875,
+ "pos_z": -21.90625,
+ "stations": "Hammond Terminal"
+ },
+ {
+ "name": "LTT 2589",
+ "pos_x": 79.84375,
+ "pos_y": -41.0625,
+ "pos_z": 12.6875,
+ "stations": "Slade Terminal,Tilman Station,Grassmann Enterprise"
+ },
+ {
+ "name": "LTT 2601",
+ "pos_x": 96.75,
+ "pos_y": -32.78125,
+ "pos_z": -53.1875,
+ "stations": "Clapperton Enterprise"
+ },
+ {
+ "name": "LTT 2622",
+ "pos_x": 69.53125,
+ "pos_y": -14.375,
+ "pos_z": -67.59375,
+ "stations": "Littlewood Landing,Pratchett Plant"
+ },
+ {
+ "name": "LTT 2623",
+ "pos_x": 83.1875,
+ "pos_y": -27.96875,
+ "pos_z": -39.15625,
+ "stations": "Brand Enterprise,Ellis Port,Love Bastion,Clarke Works,Seega Enterprise"
+ },
+ {
+ "name": "LTT 2667",
+ "pos_x": 81.46875,
+ "pos_y": -16.96875,
+ "pos_z": -58.90625,
+ "stations": "Stephenson Hub,Bretnor Hub"
+ },
+ {
+ "name": "LTT 2684",
+ "pos_x": 105.375,
+ "pos_y": -41.5,
+ "pos_z": -8.75,
+ "stations": "Lindsey Orbital,Ron Hubbard Forum,Cunningham Station,Parsons Station,Schachner Settlement,Morin Port,Levi-Montalcini Barracks,Pauling Gateway,Kondratyev City,Kooi Orbital"
+ },
+ {
+ "name": "LTT 2771",
+ "pos_x": 140.6875,
+ "pos_y": -60.6875,
+ "pos_z": 21.9375,
+ "stations": "Tiedemann Retreat"
+ },
+ {
+ "name": "LTT 2863",
+ "pos_x": 81.25,
+ "pos_y": -41.84375,
+ "pos_z": 33.21875,
+ "stations": "Tisserand Port"
+ },
+ {
+ "name": "LTT 2893",
+ "pos_x": 76.78125,
+ "pos_y": 18.28125,
+ "pos_z": -87.4375,
+ "stations": "Evans Port,Weil Reach"
+ },
+ {
+ "name": "LTT 2963",
+ "pos_x": 106.59375,
+ "pos_y": -22.1875,
+ "pos_z": -11.09375,
+ "stations": "Leestma City,Xing Hub,Nagata Landing,Dowling Point,Bohr Orbital"
+ },
+ {
+ "name": "LTT 2974",
+ "pos_x": 42.53125,
+ "pos_y": 15.65625,
+ "pos_z": -47.3125,
+ "stations": "Conrad Hub,Zhigang Port,Edwards Dock"
+ },
+ {
+ "name": "LTT 3007",
+ "pos_x": 53.96875,
+ "pos_y": 2.3125,
+ "pos_z": -26.9375,
+ "stations": "Cogswell Legacy"
+ },
+ {
+ "name": "LTT 305",
+ "pos_x": 27.6875,
+ "pos_y": -75.28125,
+ "pos_z": 21.8125,
+ "stations": "Gessi Dock,Wolf Landing,Lefschetz Hangar"
+ },
+ {
+ "name": "LTT 3290",
+ "pos_x": 92.84375,
+ "pos_y": 23.5625,
+ "pos_z": -33.78125,
+ "stations": "Grissom Dock,Kozlov Installation,Fulton Dock,Reiter Ring"
+ },
+ {
+ "name": "LTT 3488",
+ "pos_x": 57.875,
+ "pos_y": 41.65625,
+ "pos_z": -34.1875,
+ "stations": "Smith Port,McNair Survey"
+ },
+ {
+ "name": "LTT 3572",
+ "pos_x": 18.90625,
+ "pos_y": -4.21875,
+ "pos_z": 5.875,
+ "stations": "Horowitz Hub"
+ },
+ {
+ "name": "LTT 3607",
+ "pos_x": 67.4375,
+ "pos_y": -1.4375,
+ "pos_z": 11.0625,
+ "stations": "Akers Hub,Molina Dock,Chern Point,Smith Port,Fuglesang Station,Bulgakov Holdings,Jacobi Relay,Hammond Ring,Gernhardt Hub,Citroen Beacon"
+ },
+ {
+ "name": "LTT 3686",
+ "pos_x": 77.40625,
+ "pos_y": 63.5625,
+ "pos_z": -31.4375,
+ "stations": "Levchenko Station,Windt Base,Romanenko Survey"
+ },
+ {
+ "name": "LTT 3690",
+ "pos_x": 99.09375,
+ "pos_y": 22.96875,
+ "pos_z": 2.625,
+ "stations": "Cartmill City,Schweinfurth Terminal,Varthema Port,Piccard Port,Clifford Beacon,Froude Works,Kafka Installation,Skolem Lab,Bunnell Enterprise,Antonio de Andrade Depot"
+ },
+ {
+ "name": "LTT 3712",
+ "pos_x": 92.3125,
+ "pos_y": 59.875,
+ "pos_z": -23.03125,
+ "stations": "Wisoff Dock"
+ },
+ {
+ "name": "LTT 377",
+ "pos_x": 4.84375,
+ "pos_y": -63.3125,
+ "pos_z": 5.21875,
+ "stations": "Zoline Port,Garneau Survey,Macan Port,Ahern Enterprise"
+ },
+ {
+ "name": "LTT 3787",
+ "pos_x": 114.53125,
+ "pos_y": 1.1875,
+ "pos_z": 26.65625,
+ "stations": "Chern Landing,Clair Terminal,Grijalva Hub"
+ },
+ {
+ "name": "LTT 3799",
+ "pos_x": 86.65625,
+ "pos_y": 59.78125,
+ "pos_z": -17.625,
+ "stations": "O'Brien Dock,Stephens Vision,Jacquard Base,Banks Settlement"
+ },
+ {
+ "name": "LTT 3818",
+ "pos_x": 67.5625,
+ "pos_y": 55.46875,
+ "pos_z": -17.9375,
+ "stations": "Bosch Depot"
+ },
+ {
+ "name": "LTT 3919",
+ "pos_x": 50.03125,
+ "pos_y": 17.375,
+ "pos_z": 5.59375,
+ "stations": "Clairaut Refinery"
+ },
+ {
+ "name": "LTT 3981",
+ "pos_x": 96.625,
+ "pos_y": 62.6875,
+ "pos_z": 0.375,
+ "stations": "McAuley Hangar,Laird Terminal,Offutt Base,Al-Kashi Plant"
+ },
+ {
+ "name": "LTT 4042",
+ "pos_x": 100.75,
+ "pos_y": 42.03125,
+ "pos_z": 16.15625,
+ "stations": "Clark Terminal,Beckman Vision,Mondeh Station"
+ },
+ {
+ "name": "LTT 4131",
+ "pos_x": 61,
+ "pos_y": 37.84375,
+ "pos_z": 7.84375,
+ "stations": "Popper Station,Brackett Terminal,Moskowitz Ring,Budrys' Progress,Lorrah Terminal,Fox Orbital,Birkhoff Orbital,Deere Installation,Bunch Dock"
+ },
+ {
+ "name": "LTT 4150",
+ "pos_x": 61.46875,
+ "pos_y": 42.6875,
+ "pos_z": 6.96875,
+ "stations": "Fincke Terminal,Hand Hub,Brady Colony,Chapman Dock"
+ },
+ {
+ "name": "LTT 420",
+ "pos_x": 55.625,
+ "pos_y": -136.25,
+ "pos_z": 38,
+ "stations": "Denning's Claim,Truman Landing"
+ },
+ {
+ "name": "LTT 4262",
+ "pos_x": 107,
+ "pos_y": 49.40625,
+ "pos_z": 31.21875,
+ "stations": "Volterra Hangar,Narita Station,Godwin Keep,Gernsback Relay"
+ },
+ {
+ "name": "LTT 4312",
+ "pos_x": 81.375,
+ "pos_y": 53.40625,
+ "pos_z": 21.25,
+ "stations": "Midgeley Gateway"
+ },
+ {
+ "name": "LTT 4337",
+ "pos_x": 93.03125,
+ "pos_y": 35.5625,
+ "pos_z": 32.96875,
+ "stations": "Grabe City,Harbaugh Station,Zamka Terminal,Lister Port,Pascal Dock,Wang Port,Onufrienko Orbital,Horowitz City,Reightler Station,Payne Prospect,Brust Prospect,Rothman Landing"
+ },
+ {
+ "name": "LTT 4350",
+ "pos_x": 86.5625,
+ "pos_y": 5.78125,
+ "pos_z": 39.25,
+ "stations": "Komarov Orbital,Gregory Orbital,Shaver Escape"
+ },
+ {
+ "name": "LTT 4351",
+ "pos_x": 87.53125,
+ "pos_y": 54.375,
+ "pos_z": 26.03125,
+ "stations": "Gernhardt Hub,Hume Bastion,Singer Terminal,Linteris Colony"
+ },
+ {
+ "name": "LTT 4376",
+ "pos_x": 54.5,
+ "pos_y": 61.46875,
+ "pos_z": 9.625,
+ "stations": "Goulart Vision,Farrer's Inheritance"
+ },
+ {
+ "name": "LTT 4385",
+ "pos_x": 104.625,
+ "pos_y": -8.71875,
+ "pos_z": 53,
+ "stations": "Arai Dock,Adams Arsenal,Cowper Laboratory,Lalande Refinery"
+ },
+ {
+ "name": "LTT 4389",
+ "pos_x": 59.25,
+ "pos_y": 97.84375,
+ "pos_z": 3.15625,
+ "stations": "McArthur Enterprise,Fulton Beacon"
+ },
+ {
+ "name": "LTT 4428",
+ "pos_x": 75.15625,
+ "pos_y": 15.1875,
+ "pos_z": 33.34375,
+ "stations": "Levchenko Orbital,Seddon Port,Dunbar Ring,Coblentz Gateway"
+ },
+ {
+ "name": "LTT 4447",
+ "pos_x": 68.0625,
+ "pos_y": 50.40625,
+ "pos_z": 23.15625,
+ "stations": "Reamy Dock,Goulart Platform"
+ },
+ {
+ "name": "LTT 4461",
+ "pos_x": 49.625,
+ "pos_y": 44.84375,
+ "pos_z": 15.65625,
+ "stations": "Joliot-Curie Port,Sturgeon Terminal,McCormick Colony,Onufrienko Barracks"
+ },
+ {
+ "name": "LTT 4487",
+ "pos_x": 63.03125,
+ "pos_y": 30,
+ "pos_z": 26.6875,
+ "stations": "Borman Colony,Grabe's Folly,Gubarev Installation"
+ },
+ {
+ "name": "LTT 4497",
+ "pos_x": 71.0625,
+ "pos_y": 27.40625,
+ "pos_z": 31.875,
+ "stations": "Gantt Port,Merchiston Arsenal"
+ },
+ {
+ "name": "LTT 4527",
+ "pos_x": 49.9375,
+ "pos_y": 48.53125,
+ "pos_z": 17.28125,
+ "stations": "Chang-Diaz Orbital"
+ },
+ {
+ "name": "LTT 4560",
+ "pos_x": 103.125,
+ "pos_y": 32.46875,
+ "pos_z": 50.71875,
+ "stations": "Vasilyev Installation,Ryman Bastion"
+ },
+ {
+ "name": "LTT 4586",
+ "pos_x": 89.625,
+ "pos_y": -12.125,
+ "pos_z": 51.4375,
+ "stations": "Dana Dock,Gunn's Folly,Cavendish Platform"
+ },
+ {
+ "name": "LTT 4599",
+ "pos_x": 63.375,
+ "pos_y": 93.28125,
+ "pos_z": 21.15625,
+ "stations": "Phillips Gateway,Gell-Mann Gateway,Baraniecki Camp,Malzberg Installation,Cooper Gateway,Cayley Beacon"
+ },
+ {
+ "name": "LTT 464",
+ "pos_x": 22.8125,
+ "pos_y": -64.96875,
+ "pos_z": 14.8125,
+ "stations": "The Mariesh Manoevre,Hambly Platform"
+ },
+ {
+ "name": "LTT 4696",
+ "pos_x": 107,
+ "pos_y": -3.34375,
+ "pos_z": 62.9375,
+ "stations": "Arkwright Depot"
+ },
+ {
+ "name": "LTT 47",
+ "pos_x": -14.71875,
+ "pos_y": -106.15625,
+ "pos_z": 11.59375,
+ "stations": "Marcy Ring"
+ },
+ {
+ "name": "LTT 4716",
+ "pos_x": 99.53125,
+ "pos_y": 24.5625,
+ "pos_z": 56.40625,
+ "stations": "Bertin Vision,Nyberg Orbital,Cogswell Keep,Shosuke Hub"
+ },
+ {
+ "name": "LTT 4730",
+ "pos_x": 22.1875,
+ "pos_y": 30.875,
+ "pos_z": 9.84375,
+ "stations": "Gauss Landing,Clifford Dock,Kolmogorov Reformatory"
+ },
+ {
+ "name": "LTT 4772",
+ "pos_x": 50.78125,
+ "pos_y": 63.21875,
+ "pos_z": 26.53125,
+ "stations": "Minkowski City,Shelley Terminal,Norton Port,Tshang Dock,Gardner Works,Carlisle Enterprise,Laumer Hub,Reynolds Hub,MacCurdy City,Hasse Station,Abasheli Orbital,Laplace Lab"
+ },
+ {
+ "name": "LTT 4789",
+ "pos_x": 77.4375,
+ "pos_y": 79.6875,
+ "pos_z": 42.65625,
+ "stations": "Gell-Mann Terminal"
+ },
+ {
+ "name": "LTT 4835",
+ "pos_x": 73.75,
+ "pos_y": 48.09375,
+ "pos_z": 44.375,
+ "stations": "Landsteiner Outpost,Leckie Hub,Mastracchio Port"
+ },
+ {
+ "name": "LTT 4846",
+ "pos_x": 47.84375,
+ "pos_y": -8.75,
+ "pos_z": 30.125,
+ "stations": "Mullane Port,Malenchenko Observatory,Edwards Hub,Vizcaino Base,Carey Gateway"
+ },
+ {
+ "name": "LTT 4851",
+ "pos_x": 50.75,
+ "pos_y": 102.75,
+ "pos_z": 29.21875,
+ "stations": "Cabana Gateway,Priest Gateway"
+ },
+ {
+ "name": "LTT 4898",
+ "pos_x": 43.0625,
+ "pos_y": 39.71875,
+ "pos_z": 27.71875,
+ "stations": "Dana Hub,Wingqvist City,Cixin Vision,Doctorow Vision,Malzberg Relay,Bryusov Observatory"
+ },
+ {
+ "name": "LTT 4961",
+ "pos_x": 91.375,
+ "pos_y": 13.375,
+ "pos_z": 62.59375,
+ "stations": "Conway City,Greg's Legacy"
+ },
+ {
+ "name": "LTT 5013",
+ "pos_x": 85.875,
+ "pos_y": 24.09375,
+ "pos_z": 62.1875,
+ "stations": "Margulis Station,McNair Gateway,Kozeyev Settlement"
+ },
+ {
+ "name": "LTT 5053",
+ "pos_x": 71.09375,
+ "pos_y": 74.9375,
+ "pos_z": 59.4375,
+ "stations": "Ingstad Barracks,Virchow Depot,Akers Depot"
+ },
+ {
+ "name": "LTT 5058",
+ "pos_x": 79.0625,
+ "pos_y": 59.6875,
+ "pos_z": 63.65625,
+ "stations": "Kondratyev Colony,Noli Arsenal"
+ },
+ {
+ "name": "LTT 5131",
+ "pos_x": 39.28125,
+ "pos_y": 26.0625,
+ "pos_z": 32.375,
+ "stations": "Kraepelin Orbital,Spring Hub,Hunt Arena"
+ },
+ {
+ "name": "LTT 518",
+ "pos_x": 52,
+ "pos_y": -164.5625,
+ "pos_z": 29.9375,
+ "stations": "Janjetov Port,Shea Mines,Messerschmitt's Inheritance,Cowling Refinery,Merritt Port"
+ },
+ {
+ "name": "LTT 5212",
+ "pos_x": 54.1875,
+ "pos_y": 12.71875,
+ "pos_z": 43.90625,
+ "stations": "Abnett Port,Akiyama's Inheritance,Harrison Hub"
+ },
+ {
+ "name": "LTT 5237",
+ "pos_x": 40.65625,
+ "pos_y": 84.8125,
+ "pos_z": 50.53125,
+ "stations": "Sommerfeld Port,Ings Horizons,Vonnegut Penal colony"
+ },
+ {
+ "name": "LTT 5259",
+ "pos_x": 18.8125,
+ "pos_y": 59.125,
+ "pos_z": 27.625,
+ "stations": "Mitchell Colony,Birdseye Relay,Bushnell Relay"
+ },
+ {
+ "name": "LTT 5276",
+ "pos_x": 89.5625,
+ "pos_y": -11,
+ "pos_z": 69.375,
+ "stations": "Henricks Port"
+ },
+ {
+ "name": "LTT 537",
+ "pos_x": 93.90625,
+ "pos_y": -141.125,
+ "pos_z": 58.15625,
+ "stations": "Gurshtein Hub,Thiele Vision,Yamamoto Survey"
+ },
+ {
+ "name": "LTT 5419",
+ "pos_x": 30.96875,
+ "pos_y": 29.28125,
+ "pos_z": 36.78125,
+ "stations": "Libby Camp,Euclid Installation"
+ },
+ {
+ "name": "LTT 542",
+ "pos_x": 28.15625,
+ "pos_y": -140,
+ "pos_z": 13.46875,
+ "stations": "Hynek Plant,Fowler Horizons"
+ },
+ {
+ "name": "LTT 5455",
+ "pos_x": 13.0625,
+ "pos_y": 18.8125,
+ "pos_z": 17.96875,
+ "stations": "Tull Enterprise,Anthony de la Roche Barracks,Quimby Station"
+ },
+ {
+ "name": "LTT 5623",
+ "pos_x": 9.09375,
+ "pos_y": 46.28125,
+ "pos_z": 30.59375,
+ "stations": "Vries City,Metcalf Beacon"
+ },
+ {
+ "name": "LTT 5652",
+ "pos_x": 56.8125,
+ "pos_y": -9.8125,
+ "pos_z": 50.3125,
+ "stations": "Gauss Holdings"
+ },
+ {
+ "name": "LTT 5659",
+ "pos_x": 90.15625,
+ "pos_y": -40,
+ "pos_z": 67.28125,
+ "stations": "Sleator Hub,Phillpotts Installation"
+ },
+ {
+ "name": "LTT 5673",
+ "pos_x": 85.28125,
+ "pos_y": -6.25,
+ "pos_z": 80.5625,
+ "stations": "Cartwright Port,Blaha Hub,Brandenstein Market,Dozois Survey,Tarelkin Beacon,Covey Terminal,Bierce Depot,Musabayev Port"
+ },
+ {
+ "name": "LTT 569",
+ "pos_x": -16.375,
+ "pos_y": -50.125,
+ "pos_z": -13.28125,
+ "stations": "Key Dock,Baxter Reach,Hand Port,Lorrah Port"
+ },
+ {
+ "name": "LTT 5855",
+ "pos_x": 29.03125,
+ "pos_y": 45.0625,
+ "pos_z": 61.40625,
+ "stations": "Knipling Enterprise,Matteucci Settlement,Thornton Station,Forest Bastion"
+ },
+ {
+ "name": "LTT 5873",
+ "pos_x": 7.1875,
+ "pos_y": 79.5625,
+ "pos_z": 65.65625,
+ "stations": "Morgan Beacon,Eisele Camp"
+ },
+ {
+ "name": "LTT 5954",
+ "pos_x": 45.40625,
+ "pos_y": 11.4375,
+ "pos_z": 62.09375,
+ "stations": "Henize Ring,Shukor Ring,Vetulani Penal colony,Perrin Terminal,Davy Arena"
+ },
+ {
+ "name": "LTT 5964",
+ "pos_x": 43.21875,
+ "pos_y": 17.3125,
+ "pos_z": 64.96875,
+ "stations": "Witt Refinery,Anders Terminal,Resnick Station"
+ },
+ {
+ "name": "LTT 5990",
+ "pos_x": 41.15625,
+ "pos_y": 19.40625,
+ "pos_z": 65.9375,
+ "stations": "Soto Orbital,Stevens Gateway,Watt-Evans Hub,Womack Vision,Jacquard Installation,Crown Works,Bykovsky Beacon,Pashin Holdings"
+ },
+ {
+ "name": "LTT 6010",
+ "pos_x": 28.40625,
+ "pos_y": 62.0625,
+ "pos_z": 89.59375,
+ "stations": "Larson Refinery,Hunt's Progress"
+ },
+ {
+ "name": "LTT 6043",
+ "pos_x": 40.0625,
+ "pos_y": 17.03125,
+ "pos_z": 65.96875,
+ "stations": "Swainson Gateway,Shepherd Base,Brendan Enterprise,Ryumin Dock,Bhabha Enterprise"
+ },
+ {
+ "name": "LTT 606",
+ "pos_x": 0.5625,
+ "pos_y": -78.125,
+ "pos_z": -5.28125,
+ "stations": "Jeremy,Archimedes Colony,Neumann Legacy,Marianne's Journey to Arcadia"
+ },
+ {
+ "name": "LTT 6131",
+ "pos_x": 61.125,
+ "pos_y": 14.40625,
+ "pos_z": 97.09375,
+ "stations": "Denton Prospect,Effinger Refinery,Lundwall Platform"
+ },
+ {
+ "name": "LTT 6137",
+ "pos_x": 1.6875,
+ "pos_y": 40.4375,
+ "pos_z": 46.46875,
+ "stations": "Volkov Enterprise,Halsell Port,MacKellar Gateway"
+ },
+ {
+ "name": "LTT 6139",
+ "pos_x": 7.375,
+ "pos_y": 43.15625,
+ "pos_z": 57.28125,
+ "stations": "Pawelczyk Hangar,Rutherford Plant"
+ },
+ {
+ "name": "LTT 617",
+ "pos_x": -4.59375,
+ "pos_y": -133.53125,
+ "pos_z": -13.96875,
+ "stations": "Joseph Delambre Station,Land Laboratory,Person Base"
+ },
+ {
+ "name": "LTT 6216",
+ "pos_x": 37,
+ "pos_y": 38.1875,
+ "pos_z": 100.78125,
+ "stations": "Elion Settlement"
+ },
+ {
+ "name": "LTT 6255",
+ "pos_x": 36.03125,
+ "pos_y": 32.375,
+ "pos_z": 98,
+ "stations": "Mayer Enterprise"
+ },
+ {
+ "name": "LTT 6278",
+ "pos_x": 24.9375,
+ "pos_y": 26.40625,
+ "pos_z": 72.96875,
+ "stations": "Favier Installation,Bayliss Hub"
+ },
+ {
+ "name": "LTT 6299",
+ "pos_x": 70.625,
+ "pos_y": -17.34375,
+ "pos_z": 85.40625,
+ "stations": "Nagata Dock,Steele Enterprise"
+ },
+ {
+ "name": "LTT 6431",
+ "pos_x": -10.46875,
+ "pos_y": 61,
+ "pos_z": 98.03125,
+ "stations": "Cabot Vision,Shukor Terminal,Bernoulli Relay"
+ },
+ {
+ "name": "LTT 6545",
+ "pos_x": 33.90625,
+ "pos_y": 5.75,
+ "pos_z": 87.59375,
+ "stations": "Ivanishin Horizons,Laplace Installation"
+ },
+ {
+ "name": "LTT 6563",
+ "pos_x": -6.59375,
+ "pos_y": 31.78125,
+ "pos_z": 67.15625,
+ "stations": "Schottky Laboratory,Leeuwenhoek Hub,Magnus Enterprise"
+ },
+ {
+ "name": "LTT 6603",
+ "pos_x": -7.25,
+ "pos_y": 41.03125,
+ "pos_z": 98.34375,
+ "stations": "Danvers Hub,Viehbock Settlement"
+ },
+ {
+ "name": "LTT 6620",
+ "pos_x": 34.09375,
+ "pos_y": -6.21875,
+ "pos_z": 66.15625,
+ "stations": "Sharman Platform,Fuglesang City,Alexandria Keep"
+ },
+ {
+ "name": "LTT 6705",
+ "pos_x": -6.78125,
+ "pos_y": 35.59375,
+ "pos_z": 113.75,
+ "stations": "Montrose City,Shiras Hangar,Varley Works,Fozard Plant,Asher Works"
+ },
+ {
+ "name": "LTT 6710",
+ "pos_x": 5.84375,
+ "pos_y": 23.59375,
+ "pos_z": 109.15625,
+ "stations": "Otiman Landing,Onufriyenko City"
+ },
+ {
+ "name": "LTT 6714",
+ "pos_x": 35.71875,
+ "pos_y": -0.28125,
+ "pos_z": 109.34375,
+ "stations": "Lunan Station,Frobenius Terminal"
+ },
+ {
+ "name": "LTT 6772",
+ "pos_x": 54.875,
+ "pos_y": -26.59375,
+ "pos_z": 71.84375,
+ "stations": "Melvin Point,Hammond Installation,Shunn Keep"
+ },
+ {
+ "name": "LTT 6774",
+ "pos_x": 14.90625,
+ "pos_y": 1.4375,
+ "pos_z": 60.5,
+ "stations": "Kotov City,Leoniceno Orbital,Fischer Orbital,Dirac Gateway,Ramelli Terminal,Popovich Terminal"
+ },
+ {
+ "name": "LTT 6830",
+ "pos_x": 21.1875,
+ "pos_y": -2.03125,
+ "pos_z": 81.84375,
+ "stations": "Cartan Orbital,Bixby Prospect,Heiles Refinery"
+ },
+ {
+ "name": "LTT 700",
+ "pos_x": 40.78125,
+ "pos_y": -52.8125,
+ "pos_z": 23.03125,
+ "stations": "Hawke Orbital,Hiroyuki Terminal,Lagrange Enterprise,Sabine Gateway,Bachman Gateway,Morrow Hub,Macan Enterprise,RenenBellot Gateway,Shapiro Dock"
+ },
+ {
+ "name": "LTT 7069",
+ "pos_x": -24.1875,
+ "pos_y": 14.9375,
+ "pos_z": 74.28125,
+ "stations": "Nakaya Gateway,Ham Hub"
+ },
+ {
+ "name": "LTT 7126",
+ "pos_x": 57.8125,
+ "pos_y": -39.1875,
+ "pos_z": 93.125,
+ "stations": "Baydukov Dock,Phillifent Dock"
+ },
+ {
+ "name": "LTT 7138",
+ "pos_x": 10.65625,
+ "pos_y": -9.28125,
+ "pos_z": 59.875,
+ "stations": "Tanner Orbital,Schmitt Station,Buffett Station,Alexandria Relay"
+ },
+ {
+ "name": "LTT 7251",
+ "pos_x": -16.6875,
+ "pos_y": -8.09375,
+ "pos_z": 116.34375,
+ "stations": "Stephenson Horizons,Oltion Hub,Meade Penal colony"
+ },
+ {
+ "name": "LTT 7330",
+ "pos_x": 23.53125,
+ "pos_y": -27.59375,
+ "pos_z": 82.5625,
+ "stations": "Cowper Orbital,Forsskal Survey,Howard Horizons,Manning Dock"
+ },
+ {
+ "name": "LTT 7370",
+ "pos_x": 28.03125,
+ "pos_y": -26.46875,
+ "pos_z": 58.21875,
+ "stations": "Melroy Platform,Clough Keep,Arkwright Plant"
+ },
+ {
+ "name": "LTT 7421",
+ "pos_x": -23.09375,
+ "pos_y": -3.46875,
+ "pos_z": 56.9375,
+ "stations": "Lukyanenko Vision,Fancher Ring,Eudoxus Dock,Buckland Arsenal"
+ },
+ {
+ "name": "LTT 7448",
+ "pos_x": -23,
+ "pos_y": -1.09375,
+ "pos_z": 40.46875,
+ "stations": "Leiber Station,Whymper Landing,Banach Survey,Gauss Settlement"
+ },
+ {
+ "name": "LTT 7453",
+ "pos_x": -36.5625,
+ "pos_y": -7.03125,
+ "pos_z": 82.625,
+ "stations": "Gidzenko Ring,Clervoy Port,Poincare Hub,Porsche Hub,England Terminal,Teng-hui Prospect,Feoktistov Hub"
+ },
+ {
+ "name": "LTT 7488",
+ "pos_x": 32.3125,
+ "pos_y": -44.0625,
+ "pos_z": 100.375,
+ "stations": "Rubin Vision,Hambly Depot,de Andrade Base,Merritt Hub,Gutierrez Orbital"
+ },
+ {
+ "name": "LTT 7489",
+ "pos_x": 32.09375,
+ "pos_y": -43.78125,
+ "pos_z": 99.71875,
+ "stations": "Schweickart Enterprise,Thorne Hub,Meitner Survey"
+ },
+ {
+ "name": "LTT 7509",
+ "pos_x": 12.1875,
+ "pos_y": -16.03125,
+ "pos_z": 34.625,
+ "stations": "Sharman Installation,Michelson Depot,Thoreau Horizons"
+ },
+ {
+ "name": "LTT 7548",
+ "pos_x": -35.6875,
+ "pos_y": -12.21875,
+ "pos_z": 77.4375,
+ "stations": "Boltzmann Gateway,Hutton City,Alexandria Ring"
+ },
+ {
+ "name": "LTT 760",
+ "pos_x": -9.25,
+ "pos_y": -115.875,
+ "pos_z": -26.15625,
+ "stations": "Tognini Barracks,Donaldson Laboratory,Forward Landing"
+ },
+ {
+ "name": "LTT 7669",
+ "pos_x": 38.71875,
+ "pos_y": -41.71875,
+ "pos_z": 66.15625,
+ "stations": "Hooke Station,Virts Orbital,Glidden Hangar,Thesiger Holdings"
+ },
+ {
+ "name": "LTT 7701",
+ "pos_x": -15.4375,
+ "pos_y": -41.5,
+ "pos_z": 102.875,
+ "stations": "Reynolds Camp,Springer Settlement"
+ },
+ {
+ "name": "LTT 7786",
+ "pos_x": 7.96875,
+ "pos_y": -41.90625,
+ "pos_z": 77.875,
+ "stations": "Parise Gateway,Kwolek Hub,Foreman Dock,Remek Installation,Galton Depot"
+ },
+ {
+ "name": "LTT 779",
+ "pos_x": -29.90625,
+ "pos_y": -93.875,
+ "pos_z": -39.28125,
+ "stations": "Kapp Barracks,Blackwell Gateway,Melroy Terminal,Nicollier Gateway,Jemison Station,Dorsett Terminal,Fujimori Orbital,Feynman Dock"
+ },
+ {
+ "name": "LTT 7857",
+ "pos_x": 39.78125,
+ "pos_y": -39.6875,
+ "pos_z": 52.40625,
+ "stations": "Tesla Refinery,Velazquez Dock"
+ },
+ {
+ "name": "LTT 7903",
+ "pos_x": -8.09375,
+ "pos_y": -33.40625,
+ "pos_z": 60.1875,
+ "stations": "Allen Orbital,Nyberg Orbital,Lyakhov City"
+ },
+ {
+ "name": "LTT 7979",
+ "pos_x": -63.5625,
+ "pos_y": -34.875,
+ "pos_z": 76.53125,
+ "stations": "Fancher Terminal,Steinmuller Gateway,Penn Terminal"
+ },
+ {
+ "name": "LTT 8001",
+ "pos_x": -47.125,
+ "pos_y": -47.65625,
+ "pos_z": 88.09375,
+ "stations": "McBride Horizons,Filter Station,Carstensz Orbital,Payne Vista,Bulychev Station"
+ },
+ {
+ "name": "LTT 8066",
+ "pos_x": -61.03125,
+ "pos_y": -51.46875,
+ "pos_z": 89.28125,
+ "stations": "Alas City,Kondratyev Hub,Dunbar Station,Clairaut Point,Qureshi Survey"
+ },
+ {
+ "name": "LTT 8181",
+ "pos_x": -5.59375,
+ "pos_y": -20.96875,
+ "pos_z": 27.3125,
+ "stations": "Hoard Port,Wiberg Mines,Thoreau Stop"
+ },
+ {
+ "name": "LTT 8190",
+ "pos_x": 37.34375,
+ "pos_y": -48.53125,
+ "pos_z": 56.9375,
+ "stations": "Rich Terminal,Ryle Keep,Korolev Plant"
+ },
+ {
+ "name": "LTT 8260",
+ "pos_x": -3.625,
+ "pos_y": -74.25,
+ "pos_z": 88.03125,
+ "stations": "Biesbroeck Hub,de Kamp Orbital,Connes Port"
+ },
+ {
+ "name": "LTT 8410",
+ "pos_x": -2.90625,
+ "pos_y": -71.65625,
+ "pos_z": 74.3125,
+ "stations": "Piercy Dock"
+ },
+ {
+ "name": "LTT 8419",
+ "pos_x": -20.53125,
+ "pos_y": -66.34375,
+ "pos_z": 67.6875,
+ "stations": "Stableford Settlement,Aguirre Orbital,Felice Prospect,Leeuwenhoek Beacon,Cadamosto Relay"
+ },
+ {
+ "name": "LTT 8456",
+ "pos_x": 29.0625,
+ "pos_y": -42.28125,
+ "pos_z": 42.78125,
+ "stations": "Hirase Installation,Chadwick Installation"
+ },
+ {
+ "name": "LTT 8517",
+ "pos_x": 4,
+ "pos_y": -55.625,
+ "pos_z": 52.65625,
+ "stations": "Seega Dock,Hopkins Dock,Khayyam Gateway,Howe Ring,Vishweswarayya Orbital,Euclid Terminal"
+ },
+ {
+ "name": "LTT 8584",
+ "pos_x": 18.0625,
+ "pos_y": -97.0625,
+ "pos_z": 88.65625,
+ "stations": "von Zach Settlement,Lunney Dock,Gessi Base"
+ },
+ {
+ "name": "LTT 8648",
+ "pos_x": -0.75,
+ "pos_y": -66.53125,
+ "pos_z": 56.625,
+ "stations": "Peebles Dock,Harris Horizons,Hasse Penal colony,Fast Landing"
+ },
+ {
+ "name": "LTT 8657",
+ "pos_x": 4.25,
+ "pos_y": -98.0625,
+ "pos_z": 83.6875,
+ "stations": "Roe Gateway,Barcelo Relay,Adams Installation,Yanai City,Sitterly Vision"
+ },
+ {
+ "name": "LTT 8679",
+ "pos_x": -28.65625,
+ "pos_y": -32.34375,
+ "pos_z": 24,
+ "stations": "Russo Arsenal,Casper Orbital,Behnken Dock"
+ },
+ {
+ "name": "LTT 8701",
+ "pos_x": -1.65625,
+ "pos_y": -59.875,
+ "pos_z": 48.84375,
+ "stations": "Ashby City,Herzfeld Enterprise"
+ },
+ {
+ "name": "LTT 874",
+ "pos_x": 70.875,
+ "pos_y": -134.96875,
+ "pos_z": 24.78125,
+ "stations": "Young Port,Wegner Hub,Miyasaka Penal colony,Wendelin Port,Ashbrook Port,Parkinson Orbital,Bell Dock,Cooper Vision,Shoujing Hub,Lockyer Beacon"
+ },
+ {
+ "name": "LTT 8740",
+ "pos_x": -27.3125,
+ "pos_y": -98.0625,
+ "pos_z": 73,
+ "stations": "Julian Freeport,Cartan Bastion"
+ },
+ {
+ "name": "LTT 8750",
+ "pos_x": 38.15625,
+ "pos_y": -80.84375,
+ "pos_z": 69.4375,
+ "stations": "Dumont Station,Janjetov Vision,Luu Vision,Andrews Base,Caryanda Horizons,Roberts Plant"
+ },
+ {
+ "name": "LTT 8758",
+ "pos_x": -21.46875,
+ "pos_y": -84.9375,
+ "pos_z": 62.5,
+ "stations": "Alenquer Landing,McDivitt Colony"
+ },
+ {
+ "name": "LTT 8852",
+ "pos_x": 7.625,
+ "pos_y": -81.0625,
+ "pos_z": 60,
+ "stations": "Burbidge Horizons,Minkowski Station"
+ },
+ {
+ "name": "LTT 8856",
+ "pos_x": 65,
+ "pos_y": -98.5625,
+ "pos_z": 84.09375,
+ "stations": "Roskam Observatory,Wallis Market,Smirnova Orbital,Wingrove Base,Ahnert-Rohlfs Station,Maire's Folly"
+ },
+ {
+ "name": "LTT 8906",
+ "pos_x": 38.46875,
+ "pos_y": -111.1875,
+ "pos_z": 85.375,
+ "stations": "Meinel City,Impey Orbital,Flammarion Observatory,Hertzsprung Depot,King Depot,Clebsch Relay"
+ },
+ {
+ "name": "LTT 8914",
+ "pos_x": -34.21875,
+ "pos_y": -62.09375,
+ "pos_z": 36.125,
+ "stations": "Bose Refinery"
+ },
+ {
+ "name": "LTT 8968",
+ "pos_x": 15.71875,
+ "pos_y": -88,
+ "pos_z": 61.53125,
+ "stations": "Frazetta Landing,Howe Plant"
+ },
+ {
+ "name": "LTT 9028",
+ "pos_x": 17.84375,
+ "pos_y": -94.71875,
+ "pos_z": 63.5,
+ "stations": "Brothers Dock,Brill Dock,Koishikawa Vision,Szameit's Inheritance,Muhammad Ibn Battuta Depot,Merril Beacon,Gora Settlement,Chernykh Horizons"
+ },
+ {
+ "name": "LTT 9097",
+ "pos_x": 25.3125,
+ "pos_y": -82.5625,
+ "pos_z": 55.21875,
+ "stations": "Fox Gateway,Naboth Depot,Trujillo Terminal"
+ },
+ {
+ "name": "LTT 9104",
+ "pos_x": 43.5,
+ "pos_y": -69.875,
+ "pos_z": 52.875,
+ "stations": "Edwards Station,White Enterprise,Levi-Montalcini Terminal,Ricardo Works"
+ },
+ {
+ "name": "LTT 911",
+ "pos_x": 40.1875,
+ "pos_y": -92.3125,
+ "pos_z": 8.5,
+ "stations": "Brunel City,Jokester's Station,Bresnik Dock,Varley Survey,Womack Beacon"
+ },
+ {
+ "name": "LTT 9141",
+ "pos_x": 8.59375,
+ "pos_y": -100.65625,
+ "pos_z": 58.84375,
+ "stations": "Lange Mines,Alexander Enterprise,Margulies' Claim"
+ },
+ {
+ "name": "LTT 9145",
+ "pos_x": -11.53125,
+ "pos_y": -79.34375,
+ "pos_z": 40.71875,
+ "stations": "Hammond City,Lovell Dock"
+ },
+ {
+ "name": "LTT 9156",
+ "pos_x": 9.9375,
+ "pos_y": -65.65625,
+ "pos_z": 39.15625,
+ "stations": "Korolev Hub,Harding Dock,Spinrad Prospect,Hampson Barracks"
+ },
+ {
+ "name": "LTT 9164",
+ "pos_x": -36.96875,
+ "pos_y": -56.46875,
+ "pos_z": 19.34375,
+ "stations": "Debye Port"
+ },
+ {
+ "name": "LTT 9181",
+ "pos_x": 31.96875,
+ "pos_y": -61.6875,
+ "pos_z": 43.03125,
+ "stations": "Nomen Dock,Kirk Camp,Escobar Arsenal,Heyerdahl's Inheritance"
+ },
+ {
+ "name": "LTT 9246",
+ "pos_x": -53.625,
+ "pos_y": -94.6875,
+ "pos_z": 29.28125,
+ "stations": "Gabriel Enterprise,Brillant Orbital,Ackerman Terminal,Maclaurin Holdings,Flint Vision"
+ },
+ {
+ "name": "LTT 9276",
+ "pos_x": -23.25,
+ "pos_y": -105.0625,
+ "pos_z": 43.46875,
+ "stations": "Weaver Vision,Corte-Real Asylum"
+ },
+ {
+ "name": "LTT 9299",
+ "pos_x": -36.21875,
+ "pos_y": -78.46875,
+ "pos_z": 24.96875,
+ "stations": "Culbertson Ring,Herndon Prospect,Bloomfield Terminal,Virts Station,Tereshkova City,Shepherd Depot,Elwood's Progress,Daimler Ring"
+ },
+ {
+ "name": "LTT 9315",
+ "pos_x": -15.75,
+ "pos_y": -60.09375,
+ "pos_z": 22.5625,
+ "stations": "Wheelock Prospect"
+ },
+ {
+ "name": "LTT 9360",
+ "pos_x": -16.59375,
+ "pos_y": -62.6875,
+ "pos_z": 21.40625,
+ "stations": "Smeaton Orbital,Gregory Park,Deere Horizons"
+ },
+ {
+ "name": "LTT 9387",
+ "pos_x": 33.15625,
+ "pos_y": -49.21875,
+ "pos_z": 33.8125,
+ "stations": "Ferguson Gateway,Brand Hub,Hire Gateway"
+ },
+ {
+ "name": "LTT 9391",
+ "pos_x": -54.03125,
+ "pos_y": -98.8125,
+ "pos_z": 21.375,
+ "stations": "Kanwar Orbital,Bohnhoff Settlement,Chilton Dock,Petaja's Progress,Wiener Relay,Hodgkin Port"
+ },
+ {
+ "name": "LTT 9397",
+ "pos_x": -11.59375,
+ "pos_y": -83.3125,
+ "pos_z": 30.9375,
+ "stations": "Ride Holdings"
+ },
+ {
+ "name": "LTT 9455",
+ "pos_x": -15.71875,
+ "pos_y": -64.9375,
+ "pos_z": 19.53125,
+ "stations": "Goonan Station,O'Leary Vision,Gaffney Vision,Shaikh Horizons,Shaw Refinery,Lee Settlement"
+ },
+ {
+ "name": "LTT 9472",
+ "pos_x": 44.5625,
+ "pos_y": -108.9375,
+ "pos_z": 60.8125,
+ "stations": "Gonnessiat Dock,Lindstrand Orbital,Pittendreigh Relay,Reinhold Installation,Binney Settlement,McIntosh Settlement,Witt Enterprise"
+ },
+ {
+ "name": "LTT 95",
+ "pos_x": 6.78125,
+ "pos_y": -89.90625,
+ "pos_z": 17.9375,
+ "stations": "Popovich Station,Lanchester Terminal,Hewish Reach,Fairbairn Hub,Stillman Works"
+ },
+ {
+ "name": "LTT 9542",
+ "pos_x": -52.9375,
+ "pos_y": -83.28125,
+ "pos_z": 6.84375,
+ "stations": "Peterson Survey,Shonin Beacon,Bayliss Outpost"
+ },
+ {
+ "name": "LTT 9552",
+ "pos_x": 14.75,
+ "pos_y": -105.15625,
+ "pos_z": 43.25,
+ "stations": "Reichelt Orbital,Chaffee Dock,Alexander Orbital,Bartolomeu de Gusmao Gateway,Hulse Vision,Green Dock,Webb Gateway,Oxley Relay,Anvil's Inheritance,Panshin Arsenal,Coddington Dock,Arrhenius Orbital"
+ },
+ {
+ "name": "LTT 9573",
+ "pos_x": -2.09375,
+ "pos_y": -108.65625,
+ "pos_z": 35.9375,
+ "stations": "Wang Vision,Pimi Vision,Koolhaas Terminal,Wetherill Installation"
+ },
+ {
+ "name": "LTT 9577",
+ "pos_x": 68.3125,
+ "pos_y": -130.25,
+ "pos_z": 73.96875,
+ "stations": "Krenkel Settlement,Dunlop Works"
+ },
+ {
+ "name": "LTT 9593",
+ "pos_x": -39.0625,
+ "pos_y": -98.4375,
+ "pos_z": 14.9375,
+ "stations": "Ramon Dock,Caidin Stop,Bain Dock,Glashow Reach,Atwood Silo,Griggs Gateway,Lopez-Alegria Ring,Carrasco Beacon,Shinn Oasis,So-yeon Gateway,Barsanti Station,Lambert Point,Moriarty Base"
+ },
+ {
+ "name": "LTT 9605",
+ "pos_x": -2.25,
+ "pos_y": -108.46875,
+ "pos_z": 33.96875,
+ "stations": "Chertok Ring,Laurent Terminal,Dalgarno City,Alexandria City,Luyten Hub,Neugebauer Terminal,Shoemaker Terminal,Schulhof Vision,Seyfert Camp,Stasheff Hub,Kludze Ring"
+ },
+ {
+ "name": "LTT 9663",
+ "pos_x": 32.25,
+ "pos_y": -99.40625,
+ "pos_z": 44.09375,
+ "stations": "Bradley Survey,Mies van der Rohe Terminal,Neville Relay,Orbik Dock"
+ },
+ {
+ "name": "LTT 9665",
+ "pos_x": -35.5,
+ "pos_y": -62.75,
+ "pos_z": 1.625,
+ "stations": "Svavarsson Point,Narbeth Station"
+ },
+ {
+ "name": "LTT 9697",
+ "pos_x": -22.125,
+ "pos_y": -114.9375,
+ "pos_z": 20.78125,
+ "stations": "Palitzsch Terminal,Roberts Vision"
+ },
+ {
+ "name": "LTT 970",
+ "pos_x": 75.59375,
+ "pos_y": -65.28125,
+ "pos_z": 43.6875,
+ "stations": "Suzuki Enterprise,McDermott City,Bessel Dock,Quimper Silo"
+ },
+ {
+ "name": "LTT 9795",
+ "pos_x": 5.75,
+ "pos_y": -68.875,
+ "pos_z": 18.90625,
+ "stations": "Smith Port,Mayr Terminal,Herjulfsson Hub,Resnik Observatory"
+ },
+ {
+ "name": "LTT 9796",
+ "pos_x": 86.34375,
+ "pos_y": -109.9375,
+ "pos_z": 69.1875,
+ "stations": "Chretien Oudemans Arsenal,van Houten Vision"
+ },
+ {
+ "name": "LTT 9810",
+ "pos_x": 61.21875,
+ "pos_y": -99.90625,
+ "pos_z": 53.78125,
+ "stations": "Kerimov Dock,Trimble Vision,Whitford Vision,Champlain Depot,Vaucouleurs Arsenal,Gutierrez Terminal,Lopez Terminal,Friedrich Peters Vision,Minkowski Orbital,Sorayama Forum,Ferguson Terminal,Hutchinson Landing,Moffat Ring"
+ },
+ {
+ "name": "LTT 982",
+ "pos_x": 25.1875,
+ "pos_y": -85.40625,
+ "pos_z": -5.5,
+ "stations": "Nilson Installation,Lagerkvist Port,Robinson Prospect,Bayley Holdings"
+ },
+ {
+ "name": "LTT 9821",
+ "pos_x": -8.78125,
+ "pos_y": -73.59375,
+ "pos_z": 11.59375,
+ "stations": "Archimedes Installation"
+ },
+ {
+ "name": "LTT 9846",
+ "pos_x": -89.84375,
+ "pos_y": -271.96875,
+ "pos_z": 11.09375,
+ "stations": "Geoffrey's Crash"
+ },
+ {
+ "name": "LTT 9850",
+ "pos_x": -26.375,
+ "pos_y": -101.15625,
+ "pos_z": 7.5,
+ "stations": "Kroehl Dock"
+ },
+ {
+ "name": "LTT 9863",
+ "pos_x": 24.65625,
+ "pos_y": -117.03125,
+ "pos_z": 36.90625,
+ "stations": "Lynden-Bell Terminal,Pei Orbital"
+ },
+ {
+ "name": "Lu Bei",
+ "pos_x": 111.4375,
+ "pos_y": -60.9375,
+ "pos_z": 26.34375,
+ "stations": "Sutter Settlement"
+ },
+ {
+ "name": "Lu Benzai",
+ "pos_x": -101,
+ "pos_y": 122.09375,
+ "pos_z": 5.625,
+ "stations": "Savinykh Landing,Coleman Survey"
+ },
+ {
+ "name": "Lu Bese",
+ "pos_x": -125.5625,
+ "pos_y": 30.53125,
+ "pos_z": 107.4375,
+ "stations": "Aikin Mines,Cartier Camp,Klink's Folly"
+ },
+ {
+ "name": "Lu Bosate",
+ "pos_x": -64.5,
+ "pos_y": -151.96875,
+ "pos_z": -23.375,
+ "stations": "Wheelock Gateway,Sekowski Arsenal"
+ },
+ {
+ "name": "Lu Di",
+ "pos_x": 167.90625,
+ "pos_y": -164.8125,
+ "pos_z": 76.25,
+ "stations": "Dominique Terminal"
+ },
+ {
+ "name": "Lu Dimos",
+ "pos_x": -80.53125,
+ "pos_y": -12.6875,
+ "pos_z": 131.15625,
+ "stations": "Baird City,Mitchell's Claim,Napier Point"
+ },
+ {
+ "name": "Lu Dis",
+ "pos_x": 93.625,
+ "pos_y": -11.8125,
+ "pos_z": -130.03125,
+ "stations": "Abel Hub,Menezes Mines,Norton Settlement,Citi Horizons,Paulmier de Gonneville Reach"
+ },
+ {
+ "name": "Lu Disc",
+ "pos_x": -129.46875,
+ "pos_y": -18.78125,
+ "pos_z": -35.75,
+ "stations": "Trevithick Point,Zhigang Landing"
+ },
+ {
+ "name": "Lu Dong Bin",
+ "pos_x": -10.625,
+ "pos_y": 11.21875,
+ "pos_z": 162.71875,
+ "stations": "Daniel Prospect"
+ },
+ {
+ "name": "Lu Dongbin",
+ "pos_x": 92.6875,
+ "pos_y": 5.90625,
+ "pos_z": 45.1875,
+ "stations": "Luiken Settlement"
+ },
+ {
+ "name": "Lu Dongia",
+ "pos_x": 99.5,
+ "pos_y": -62.09375,
+ "pos_z": -65.5,
+ "stations": "Citroen Dock,Soddy Ring,Fraley Enterprise,Rutherford Orbital"
+ },
+ {
+ "name": "Lu Hsiensei",
+ "pos_x": 7.125,
+ "pos_y": -121.75,
+ "pos_z": -34.59375,
+ "stations": "Israel Base,Baker Vision"
+ },
+ {
+ "name": "Lu Pah",
+ "pos_x": 77.71875,
+ "pos_y": -118.21875,
+ "pos_z": 2.9375,
+ "stations": "Rothfuss Mines"
+ },
+ {
+ "name": "Lu Pani",
+ "pos_x": 90.21875,
+ "pos_y": -213.53125,
+ "pos_z": -32.09375,
+ "stations": "Davies Gateway,Asher Base"
+ },
+ {
+ "name": "Lu Pao",
+ "pos_x": -154.6875,
+ "pos_y": 45.0625,
+ "pos_z": -1.03125,
+ "stations": "Swift Vision,Burnham Landing,Cardano Ring,Semeonis Landing,Heceta Survey"
+ },
+ {
+ "name": "Lu Pardan",
+ "pos_x": -28.34375,
+ "pos_y": 7.78125,
+ "pos_z": -115.71875,
+ "stations": "Bolotov Station,Sacco Port,Krylov Penal colony"
+ },
+ {
+ "name": "Lu Pel",
+ "pos_x": -10.8125,
+ "pos_y": 47.0625,
+ "pos_z": 152.09375,
+ "stations": "Haipeng Hub"
+ },
+ {
+ "name": "Lu Pinda",
+ "pos_x": 87.28125,
+ "pos_y": -29.3125,
+ "pos_z": 97.75,
+ "stations": "Clifton Base,Byrd Refinery"
+ },
+ {
+ "name": "Lu Tiegari",
+ "pos_x": 98.40625,
+ "pos_y": -199.0625,
+ "pos_z": -51.5,
+ "stations": "Arnold Mines"
+ },
+ {
+ "name": "Lu Tienses",
+ "pos_x": -77.28125,
+ "pos_y": -48.84375,
+ "pos_z": 152.53125,
+ "stations": "Wright Beacon,Oxley Legacy"
+ },
+ {
+ "name": "Lu Tun",
+ "pos_x": 75.4375,
+ "pos_y": -23.21875,
+ "pos_z": -141.34375,
+ "stations": "Collins Reach,Killough Outpost"
+ },
+ {
+ "name": "Lu Tungatya",
+ "pos_x": 130.03125,
+ "pos_y": 86.375,
+ "pos_z": -6.46875,
+ "stations": "Gwynn's Inheritance"
+ },
+ {
+ "name": "LU Velorum",
+ "pos_x": 49.875,
+ "pos_y": 5.875,
+ "pos_z": 4.3125,
+ "stations": "Miletus Station,Kavandi Barracks,Card Enterprise,Zhen Observatory,Alten Gateway"
+ },
+ {
+ "name": "Lu Wang",
+ "pos_x": -79.03125,
+ "pos_y": 51.8125,
+ "pos_z": 45.53125,
+ "stations": "Smith Hub,Rukavishnikov Dock,Farkas Orbital,Gell-Mann Terminal,Amnuel Gateway,Poisson's Folly"
+ },
+ {
+ "name": "Lu Wanja",
+ "pos_x": -77.96875,
+ "pos_y": -66.34375,
+ "pos_z": -85.75,
+ "stations": "Lonchakov Ring,Carr Enterprise,Brunel Orbital,Carpini Vision,Stefansson Penal colony"
+ },
+ {
+ "name": "Lu Wu Guan",
+ "pos_x": 4.8125,
+ "pos_y": -110.5,
+ "pos_z": 113.34375,
+ "stations": "Ortiz Moreno Hub,Kizawa Terminal,Poincare Holdings"
+ },
+ {
+ "name": "Lu Xiangu",
+ "pos_x": 80.3125,
+ "pos_y": -139.5,
+ "pos_z": 142.0625,
+ "stations": "Krenkel Vision,Al Sufi Hub,Hariot Port,Verne Settlement"
+ },
+ {
+ "name": "Lu Xians",
+ "pos_x": 61.8125,
+ "pos_y": -103.6875,
+ "pos_z": 84.96875,
+ "stations": "Lilienthal Arena"
+ },
+ {
+ "name": "Lu Xianses",
+ "pos_x": 40.6875,
+ "pos_y": 29.6875,
+ "pos_z": 106,
+ "stations": "Tesla Hangar"
+ },
+ {
+ "name": "Lu Xiao",
+ "pos_x": 101.625,
+ "pos_y": 107.25,
+ "pos_z": -26.46875,
+ "stations": "Crown Terminal,Lasswitz Hub"
+ },
+ {
+ "name": "Lu Yai",
+ "pos_x": -42.40625,
+ "pos_y": -141.25,
+ "pos_z": -3.34375,
+ "stations": "Kononenko Prospect,Timofeyevich Depot,Pawelczyk Survey"
+ },
+ {
+ "name": "Lu Yupik",
+ "pos_x": 21.53125,
+ "pos_y": -19.21875,
+ "pos_z": 4.25,
+ "stations": "Edison Settlement,Bagian Survey"
+ },
+ {
+ "name": "Luan Yun Di",
+ "pos_x": 36.6875,
+ "pos_y": -19.75,
+ "pos_z": 57.96875,
+ "stations": "Gaiman Dock,Drzewiecki Orbital,Morris Enterprise"
+ },
+ {
+ "name": "Luana",
+ "pos_x": 17.25,
+ "pos_y": -133.5,
+ "pos_z": -98.75,
+ "stations": "Coddington Oasis,Shajn Station,Fernandez Plant"
+ },
+ {
+ "name": "Luang Te",
+ "pos_x": 77.875,
+ "pos_y": 70.84375,
+ "pos_z": -106.1875,
+ "stations": "Britnev Mine,Beliaev Depot,Fawcett's Folly"
+ },
+ {
+ "name": "Luang Yans",
+ "pos_x": 101.78125,
+ "pos_y": 23.1875,
+ "pos_z": -131.4375,
+ "stations": "Coppel Enterprise,Andrews Co-operative"
+ },
+ {
+ "name": "Lubal",
+ "pos_x": 65.90625,
+ "pos_y": -79.34375,
+ "pos_z": -109.9375,
+ "stations": "Salk Colony"
+ },
+ {
+ "name": "Lubaluhet",
+ "pos_x": -87.90625,
+ "pos_y": 58.53125,
+ "pos_z": 29.15625,
+ "stations": "Dobrovolski Orbital,Collins Orbital"
+ },
+ {
+ "name": "Lucab Ku",
+ "pos_x": -132.75,
+ "pos_y": 48.28125,
+ "pos_z": -41.4375,
+ "stations": "Whitney Works,Wohler Dock,Goonan's Folly"
+ },
+ {
+ "name": "Lucadrus",
+ "pos_x": -17.0625,
+ "pos_y": -158,
+ "pos_z": -59.59375,
+ "stations": "Harrington Settlement,Friedman Park,Parker Ring,Vasilyev Penal colony,Kerr Vision"
+ },
+ {
+ "name": "Luch",
+ "pos_x": 204.5625,
+ "pos_y": -168.875,
+ "pos_z": -12.09375,
+ "stations": "Bell Enterprise,Salpeter Mines,Dahm Terminal,Woolley Penal colony"
+ },
+ {
+ "name": "Luchantauo",
+ "pos_x": -63.0625,
+ "pos_y": 19.21875,
+ "pos_z": 117.9375,
+ "stations": "Tanner Prospect,Dashiell Colony"
+ },
+ {
+ "name": "Lucharn",
+ "pos_x": -92.1875,
+ "pos_y": -29.75,
+ "pos_z": -41.78125,
+ "stations": "Gresley Point,Halley Vision"
+ },
+ {
+ "name": "Luchoer",
+ "pos_x": 73.125,
+ "pos_y": -136.3125,
+ "pos_z": 4.96875,
+ "stations": "Truman Reformatory"
+ },
+ {
+ "name": "Luchtaine",
+ "pos_x": -9523.3125,
+ "pos_y": -914.46875,
+ "pos_z": 19825.90625,
+ "stations": "Moore's Charm,Neugebauer Mines,The Brig"
+ },
+ {
+ "name": "Luchu",
+ "pos_x": 85.15625,
+ "pos_y": -46.65625,
+ "pos_z": -2.125,
+ "stations": "Neumann Enterprise,Walheim Enterprise,Onufrienko Outpost"
+ },
+ {
+ "name": "Luchunza",
+ "pos_x": -89.46875,
+ "pos_y": -103.65625,
+ "pos_z": -64.46875,
+ "stations": "Krikalev Base,Elder Settlement"
+ },
+ {
+ "name": "Lugger",
+ "pos_x": 100.15625,
+ "pos_y": 19.3125,
+ "pos_z": 25.46875,
+ "stations": "Caryanda Enterprise,Brahe Installation,Wagner Ring,Scully-Power Enterprise,Killough Port,Stackpole City,Martins Enterprise,Matheson Enterprise,Ayerdhal Port,Pinto Palace"
+ },
+ {
+ "name": "Luggerates",
+ "pos_x": -106.8125,
+ "pos_y": 42.0625,
+ "pos_z": -63.9375,
+ "stations": "Gaspar de Portola Prospect,Morrison Prospect,Barcelos' Inheritance"
+ },
+ {
+ "name": "Luggsa",
+ "pos_x": 151.0625,
+ "pos_y": -74.40625,
+ "pos_z": 102.53125,
+ "stations": "Parkinson Colony"
+ },
+ {
+ "name": "Lugh",
+ "pos_x": 44.65625,
+ "pos_y": -5.71875,
+ "pos_z": 27.46875,
+ "stations": "Cavendish Ring,Seega Port,Balandin Gateway,Knight Dock,Hartsfield Market,Meyrink Depot,Read Gateway,Chun Works,Prunariu Orbital,MacLean Hub,Adamson Enterprise"
+ },
+ {
+ "name": "Lugievachis",
+ "pos_x": 36.34375,
+ "pos_y": -232.375,
+ "pos_z": 53.21875,
+ "stations": "Alenquer Hub,Cherry Stop"
+ },
+ {
+ "name": "Lugievisci",
+ "pos_x": 164.09375,
+ "pos_y": -172.875,
+ "pos_z": 56.96875,
+ "stations": "Foss Dock"
+ },
+ {
+ "name": "Lugiu Di",
+ "pos_x": -73.71875,
+ "pos_y": -130.65625,
+ "pos_z": 18.1875,
+ "stations": "Lukyanenko Prison,Williamson's Progress,Tilley Dock"
+ },
+ {
+ "name": "Lugiu Dieva",
+ "pos_x": 110.9375,
+ "pos_y": -33.75,
+ "pos_z": 16.34375,
+ "stations": "Eisele Port"
+ },
+ {
+ "name": "Lugul",
+ "pos_x": -69.125,
+ "pos_y": 70.8125,
+ "pos_z": -42.34375,
+ "stations": "Yamazaki Enterprise,Gregory Vision,Godwin Enterprise"
+ },
+ {
+ "name": "Luguls",
+ "pos_x": 138.875,
+ "pos_y": -80.375,
+ "pos_z": 37.3125,
+ "stations": "Kushida Ring,Takamizawa Ring,Rae Base,Elst Hub,Sellings Installation"
+ },
+ {
+ "name": "Lugupa",
+ "pos_x": 58.71875,
+ "pos_y": 114.46875,
+ "pos_z": 71.03125,
+ "stations": "Oxley Platform"
+ },
+ {
+ "name": "Luhman 16",
+ "pos_x": 6.3125,
+ "pos_y": 0.59375,
+ "pos_z": 1.71875,
+ "stations": "Jenner Orbital,Heisenberg Colony,Edison Hub"
+ },
+ {
+ "name": "Luk Xing",
+ "pos_x": -84.53125,
+ "pos_y": 35.1875,
+ "pos_z": 123.03125,
+ "stations": "Lopez de Legazpi Orbital,Kratman Terminal,Samuda Horizons,Dickson Orbital"
+ },
+ {
+ "name": "Lulua",
+ "pos_x": 120.625,
+ "pos_y": 20.28125,
+ "pos_z": 22.96875,
+ "stations": "Cassidy Orbital,Panshin Barracks,Nearchus Enterprise"
+ },
+ {
+ "name": "Lulumbla",
+ "pos_x": 21.0625,
+ "pos_y": 69.15625,
+ "pos_z": 150.5625,
+ "stations": "Clauss Settlement"
+ },
+ {
+ "name": "Lulunguni",
+ "pos_x": -77.40625,
+ "pos_y": -49.65625,
+ "pos_z": 96.71875,
+ "stations": "Kizim Colony,Worden Station,Lovelace Terminal,Hardwick Forum"
+ },
+ {
+ "name": "Lulus",
+ "pos_x": 47.4375,
+ "pos_y": -206.0625,
+ "pos_z": 84.78125,
+ "stations": "Riazuddin Station,Dalgarno Dock,Urata Hub,Kaiser Port,Oshima Station,Baffin Palace,Kummer Survey,Biggle Laboratory,Czerneda Base"
+ },
+ {
+ "name": "Luluwala",
+ "pos_x": 58.53125,
+ "pos_y": -69.625,
+ "pos_z": 36.625,
+ "stations": "Blenkinsop Settlement"
+ },
+ {
+ "name": "Luma",
+ "pos_x": -40.84375,
+ "pos_y": -133.625,
+ "pos_z": 58,
+ "stations": "Binney City,Folland Vision,Dubyago Hub,Dawes Prospect"
+ },
+ {
+ "name": "Lumanans",
+ "pos_x": 56.96875,
+ "pos_y": -48.28125,
+ "pos_z": 129.65625,
+ "stations": "Leoniceno Refinery,Pirsan Dock"
+ },
+ {
+ "name": "Lumanata",
+ "pos_x": 90.25,
+ "pos_y": -123.84375,
+ "pos_z": 48.09375,
+ "stations": "Matthaus Olbers Colony,Littrow Port"
+ },
+ {
+ "name": "Lumaragro",
+ "pos_x": -162.28125,
+ "pos_y": 33.0625,
+ "pos_z": -92,
+ "stations": "Eisenstein Prospect,Effinger Point,Silverberg Camp"
+ },
+ {
+ "name": "Lumastya",
+ "pos_x": 81.8125,
+ "pos_y": -154.53125,
+ "pos_z": 63.1875,
+ "stations": "Suzuki Terminal,Tikhonravov Vision"
+ },
+ {
+ "name": "Lumba",
+ "pos_x": -35.28125,
+ "pos_y": -89.59375,
+ "pos_z": -33.375,
+ "stations": "Hottot's Inheritance,Tombaugh Station,Fujimori Orbital,Cseszneky Settlement"
+ },
+ {
+ "name": "Lumbawetiac",
+ "pos_x": 182.0625,
+ "pos_y": -146.0625,
+ "pos_z": -7.5,
+ "stations": "Foerster Depot,Zwicky Keep"
+ },
+ {
+ "name": "Lumbla",
+ "pos_x": 44.65625,
+ "pos_y": -3.40625,
+ "pos_z": -88.8125,
+ "stations": "Perrin Ring,Hui Penal colony"
+ },
+ {
+ "name": "Lun Du",
+ "pos_x": -118.84375,
+ "pos_y": -126.53125,
+ "pos_z": -9.53125,
+ "stations": "Barnwell Point"
+ },
+ {
+ "name": "Lunda",
+ "pos_x": 121.46875,
+ "pos_y": 35.125,
+ "pos_z": 103.15625,
+ "stations": "Pierres Terminal,Huxley Holdings,Gerlache Settlement"
+ },
+ {
+ "name": "Lundji",
+ "pos_x": 56.4375,
+ "pos_y": 65.28125,
+ "pos_z": 121.09375,
+ "stations": "Singer Hub,MacKellar Orbital,Leibniz's Folly,Ivins Hub"
+ },
+ {
+ "name": "Lunduwalaya",
+ "pos_x": 2.59375,
+ "pos_y": -57.9375,
+ "pos_z": 127.84375,
+ "stations": "Volterra's Folly,Cabrera Mines,Blenkinsop Platform"
+ },
+ {
+ "name": "Lung",
+ "pos_x": 3.71875,
+ "pos_y": -6.53125,
+ "pos_z": -13.53125,
+ "stations": "Piccard Plant,Hopkins Landing,Qureshi Hangar,McAllaster's Folly,Harvey Station"
+ },
+ {
+ "name": "Lunga",
+ "pos_x": 20.75,
+ "pos_y": -99.78125,
+ "pos_z": -120.3125,
+ "stations": "Cabral Hub,Mieville Station"
+ },
+ {
+ "name": "Lungundani",
+ "pos_x": -119.8125,
+ "pos_y": -47.25,
+ "pos_z": -20.40625,
+ "stations": "Regiomontanus Orbital,O'Connor Installation"
+ },
+ {
+ "name": "Lunguni",
+ "pos_x": -77.1875,
+ "pos_y": -123.84375,
+ "pos_z": -107.375,
+ "stations": "Doyle Landing,Bendell Terminal,Macedo Freeport,Compton Prospect,Rescue Ship - Doyle Landing"
+ },
+ {
+ "name": "Luo Wang",
+ "pos_x": 62.84375,
+ "pos_y": -123,
+ "pos_z": -97.21875,
+ "stations": "Addams Camp"
+ },
+ {
+ "name": "Lupheng",
+ "pos_x": 107.96875,
+ "pos_y": 45.46875,
+ "pos_z": -30.84375,
+ "stations": "Borlaug Mine,Wisoff Base"
+ },
+ {
+ "name": "Luphinn",
+ "pos_x": 160.5625,
+ "pos_y": -68.09375,
+ "pos_z": 96.53125,
+ "stations": "Polikarpov Works,Zwicky Stop,van Vogt Hub"
+ },
+ {
+ "name": "Luphis",
+ "pos_x": 139.71875,
+ "pos_y": -163.09375,
+ "pos_z": 99.5,
+ "stations": "van Rhijn Dock,Brouwer Platform"
+ },
+ {
+ "name": "Lushai",
+ "pos_x": -93.5,
+ "pos_y": 6.78125,
+ "pos_z": 91.96875,
+ "stations": "Bramah Dock"
+ },
+ {
+ "name": "Lushertha",
+ "pos_x": -7.09375,
+ "pos_y": 2.25,
+ "pos_z": -19.96875,
+ "stations": "de Caminha Station,Laumer Hub,Cook Ring,Tshang Station,Butcher Keep,Lewis Vision,Asher Beacon,Zindell Beacon"
+ },
+ {
+ "name": "Lushu",
+ "pos_x": -7.9375,
+ "pos_y": -90.6875,
+ "pos_z": 0.40625,
+ "stations": "Dyr Ring,Delany Beacon,Bloch Port,Paulo da Gama Port,Krupkat's Folly"
+ },
+ {
+ "name": "Luso",
+ "pos_x": -120.375,
+ "pos_y": 24.3125,
+ "pos_z": 31.15625,
+ "stations": "Lockhart Orbital,Diesel Port,Arber Settlement,Fincke Point,Crichton Laboratory"
+ },
+ {
+ "name": "Lusonda",
+ "pos_x": -34.28125,
+ "pos_y": -67.03125,
+ "pos_z": 45.125,
+ "stations": "Lee Orbital"
+ },
+ {
+ "name": "Lusoneshas",
+ "pos_x": 50.25,
+ "pos_y": -167.65625,
+ "pos_z": -12.3125,
+ "stations": "Arnold Terminal,Lasswitz Installation,Bond Terminal"
+ },
+ {
+ "name": "Lusong",
+ "pos_x": 117.78125,
+ "pos_y": -106.53125,
+ "pos_z": 96.46875,
+ "stations": "Mainzer Colony"
+ },
+ {
+ "name": "Lusonne",
+ "pos_x": -43.375,
+ "pos_y": -17.25,
+ "pos_z": 116.25,
+ "stations": "Clairaut City,Kimberlin Station,Tevis Port,Thurston Landing,Tolstoi Settlement,Rowley Station,Flinders City,Hodgkinson Holdings,Hughes Survey"
+ },
+ {
+ "name": "Lusonnunga",
+ "pos_x": 160.34375,
+ "pos_y": -103.90625,
+ "pos_z": 56.78125,
+ "stations": "Edmondson Gateway,Schmidt Orbital"
+ },
+ {
+ "name": "Lutnahua",
+ "pos_x": -45.40625,
+ "pos_y": -53.15625,
+ "pos_z": 139.1875,
+ "stations": "Stapledon Dock,Herndon Depot,Roggeveen Terminal,Pond Terminal"
+ },
+ {
+ "name": "Lutni",
+ "pos_x": 89.875,
+ "pos_y": -77.4375,
+ "pos_z": -14.09375,
+ "stations": "Izumikawa Settlement,Delaunay Orbital,Adelman Terminal,Mobius' Folly"
+ },
+ {
+ "name": "Luvalla",
+ "pos_x": -110.78125,
+ "pos_y": 35.34375,
+ "pos_z": -107.9375,
+ "stations": "Ejeta Station,Wingqvist Ring,Nowak City,Beatty Horizons"
+ },
+ {
+ "name": "Luvana",
+ "pos_x": 99.3125,
+ "pos_y": 58.46875,
+ "pos_z": -12.59375,
+ "stations": "Grzimek Mines,Klimuk Enterprise"
+ },
+ {
+ "name": "Lux Caeli",
+ "pos_x": -9489.96875,
+ "pos_y": -911.71875,
+ "pos_z": 19818.03125,
+ "stations": "Tristan's Rest"
+ },
+ {
+ "name": "Luyten 145-141",
+ "pos_x": 13.4375,
+ "pos_y": -0.8125,
+ "pos_z": 6.65625,
+ "stations": "Euclid Station,Kerr Survey"
+ },
+ {
+ "name": "Luyten 205-128",
+ "pos_x": 7.59375,
+ "pos_y": -4.9375,
+ "pos_z": 16.71875,
+ "stations": "Shuttleworth Holdings,H. G. Wells Hub,Robins High,Kotzebue Works"
+ },
+ {
+ "name": "Luyten 347-14",
+ "pos_x": 2.21875,
+ "pos_y": -7.53125,
+ "pos_z": 16.8125,
+ "stations": "Gerrold Prospect,Mendel Palace"
+ },
+ {
+ "name": "Luyten 674-15",
+ "pos_x": 16.71875,
+ "pos_y": 2.28125,
+ "pos_z": -9.125,
+ "stations": "Sinclair Relay,Nobleport,Tayler Platform,Garden Vista,Fernandes de Queiros Exchange"
+ },
+ {
+ "name": "Luyten's Star",
+ "pos_x": 6.5625,
+ "pos_y": 2.34375,
+ "pos_z": -10.25,
+ "stations": "Ashby City,McNair Gateway,Nikitin Silo,Payson Installation"
+ },
+ {
+ "name": "Lwalak",
+ "pos_x": -39.28125,
+ "pos_y": -133.53125,
+ "pos_z": 97.0625,
+ "stations": "Zarnecki Depot"
+ },
+ {
+ "name": "Lwalama",
+ "pos_x": 20.625,
+ "pos_y": -85.5625,
+ "pos_z": 59.875,
+ "stations": "Argelander Vision,Langley City,Wachmann Station,Gasparis Station"
+ },
+ {
+ "name": "Lwali",
+ "pos_x": 1.03125,
+ "pos_y": -153.9375,
+ "pos_z": -28.8125,
+ "stations": "Narbeth Dock"
+ },
+ {
+ "name": "Lwalkinus",
+ "pos_x": 144.25,
+ "pos_y": -129.6875,
+ "pos_z": 124.25,
+ "stations": "Potocnik Depot"
+ },
+ {
+ "name": "Lwen",
+ "pos_x": -50.34375,
+ "pos_y": -28.3125,
+ "pos_z": 87.53125,
+ "stations": "Oliver Enterprise,McIntyre's Folly,Phillips Terminal,Hippalus Gateway,von Helmont Silo"
+ },
+ {
+ "name": "Lwena",
+ "pos_x": 141.78125,
+ "pos_y": -148.4375,
+ "pos_z": -3.625,
+ "stations": "Paczynski Station,Lintott Dock,Vasilyev Holdings,Young Terminal"
+ },
+ {
+ "name": "Lwendecht",
+ "pos_x": 94.0625,
+ "pos_y": -255.21875,
+ "pos_z": 53.375,
+ "stations": "Wilhelm Enterprise,Bennett Vision,Stiles Orbital,Cook Refinery,Marriott Depot"
+ },
+ {
+ "name": "Lycanthrope",
+ "pos_x": -9506.4375,
+ "pos_y": -944.125,
+ "pos_z": 19795.0625,
+ "stations": "The Crypt"
+ },
+ {
+ "name": "Lyncis Sector DB-X c1-9",
+ "pos_x": -67.4375,
+ "pos_y": 121.96875,
+ "pos_z": -132.75,
+ "stations": "VanderMeer Legacy"
+ },
+ {
+ "name": "Lyncis Sector DL-Y d62",
+ "pos_x": -40.8125,
+ "pos_y": 78.5,
+ "pos_z": -140.9375,
+ "stations": "Auer Barracks"
+ },
+ {
+ "name": "Lyncis Sector DL-Y d78",
+ "pos_x": -51.5,
+ "pos_y": 81,
+ "pos_z": -113.1875,
+ "stations": "Lerner Point,Shull Terminal"
+ },
+ {
+ "name": "Lyncis Sector DL-Y d88",
+ "pos_x": -28.9375,
+ "pos_y": 104.65625,
+ "pos_z": -129.4375,
+ "stations": "Norman's Progress,Wylie Depot"
+ },
+ {
+ "name": "Lyncis Sector DQ-Y c15",
+ "pos_x": -6.3125,
+ "pos_y": 88.5,
+ "pos_z": -154.34375,
+ "stations": "Zindell Laboratory,Piccard Escape"
+ },
+ {
+ "name": "Lyncis Sector DQ-Y c7",
+ "pos_x": -13.9375,
+ "pos_y": 80.71875,
+ "pos_z": -171.28125,
+ "stations": "Maler's Progress"
+ },
+ {
+ "name": "Lyncis Sector DQ-Y c9",
+ "pos_x": -16.78125,
+ "pos_y": 55.03125,
+ "pos_z": -171.5625,
+ "stations": "Kimberlin's Inheritance"
+ },
+ {
+ "name": "Lyncis Sector EB-X c1-11",
+ "pos_x": -33.21875,
+ "pos_y": 95.5,
+ "pos_z": -112.4375,
+ "stations": "Payton's Inheritance"
+ },
+ {
+ "name": "Lyncis Sector EB-X c1-8",
+ "pos_x": -27.90625,
+ "pos_y": 105.4375,
+ "pos_z": -122.71875,
+ "stations": "Jeury Hub"
+ },
+ {
+ "name": "Lyncis Sector EG-Y d124",
+ "pos_x": -110.21875,
+ "pos_y": 50.34375,
+ "pos_z": -130.65625,
+ "stations": "Martin Forum"
+ },
+ {
+ "name": "Lyncis Sector EL-Y c19",
+ "pos_x": -37.09375,
+ "pos_y": 42.28125,
+ "pos_z": -155.21875,
+ "stations": "Burgess Oasis"
+ },
+ {
+ "name": "Lyncis Sector EL-Y c5",
+ "pos_x": -31.4375,
+ "pos_y": 20.125,
+ "pos_z": -169.84375,
+ "stations": "Webb Laboratory"
+ },
+ {
+ "name": "Lyncis Sector EW-W c1-13",
+ "pos_x": -106.09375,
+ "pos_y": 74.8125,
+ "pos_z": -111.53125,
+ "stations": "Cousteau's Claim,Ledyard Arena"
+ },
+ {
+ "name": "Lyncis Sector EW-W c1-6",
+ "pos_x": -113.1875,
+ "pos_y": 78.8125,
+ "pos_z": -135.625,
+ "stations": "Thompson Reach"
+ },
+ {
+ "name": "Lyncis Sector FG-Y d85",
+ "pos_x": -28.28125,
+ "pos_y": 40.46875,
+ "pos_z": -158.5,
+ "stations": "Wul Installation,Niven's Folly,Heck's Folly"
+ },
+ {
+ "name": "Lyncis Sector FG-Y d87",
+ "pos_x": -31.03125,
+ "pos_y": 52.71875,
+ "pos_z": -150.4375,
+ "stations": "Hamilton Relay,Hamilton Penal colony"
+ },
+ {
+ "name": "Lyncis Sector GR-W c1-4",
+ "pos_x": -107.09375,
+ "pos_y": 36.625,
+ "pos_z": -109.40625,
+ "stations": "Lindbohm Laboratory,Shaikh Installation"
+ },
+ {
+ "name": "Lyncis Sector HH-V c2-12",
+ "pos_x": -67.0625,
+ "pos_y": 107.125,
+ "pos_z": -93.15625,
+ "stations": "Birmingham Beacon"
+ },
+ {
+ "name": "Lyncis Sector JR-W c1-13",
+ "pos_x": -4.53125,
+ "pos_y": 54.125,
+ "pos_z": -143,
+ "stations": "Gibbs Laboratory"
+ },
+ {
+ "name": "Lyncis Sector KM-V b2-3",
+ "pos_x": -13.5625,
+ "pos_y": 85.90625,
+ "pos_z": -162.0625,
+ "stations": "Bulychev Laboratory"
+ },
+ {
+ "name": "Lyncis Sector LC-V b2-1",
+ "pos_x": -76.03125,
+ "pos_y": 42.28125,
+ "pos_z": -162.0625,
+ "stations": "Terry Landing"
+ },
+ {
+ "name": "Lyncis Sector MC-V b2-1",
+ "pos_x": -54.625,
+ "pos_y": 43.1875,
+ "pos_z": -156.375,
+ "stations": "Baudin Landing,Macedo Camp"
+ },
+ {
+ "name": "Lyncis Sector MH-V b2-3",
+ "pos_x": -7.625,
+ "pos_y": 59.9375,
+ "pos_z": -162.21875,
+ "stations": "Makeev Plant"
+ },
+ {
+ "name": "Lyncis Sector MN-T b3-1",
+ "pos_x": -88.46875,
+ "pos_y": 68.375,
+ "pos_z": -136.65625,
+ "stations": "Conway Keep"
+ },
+ {
+ "name": "Lyncis Sector OI-T b3-2",
+ "pos_x": -101.53125,
+ "pos_y": 49.46875,
+ "pos_z": -133.1875,
+ "stations": "Malchiodi Base"
+ },
+ {
+ "name": "Lyncis Sector OX-U b2-4",
+ "pos_x": -49.5625,
+ "pos_y": 19.125,
+ "pos_z": -146.0625,
+ "stations": "Cremona Vision,Pettit Base"
+ },
+ {
+ "name": "Lyncis Sector PD-T b3-2",
+ "pos_x": -121.875,
+ "pos_y": 27.53125,
+ "pos_z": -138.21875,
+ "stations": "Marlowe's Inheritance"
+ },
+ {
+ "name": "Lyncis Sector PY-R b4-2",
+ "pos_x": -74.25,
+ "pos_y": 81.96875,
+ "pos_z": -112.0625,
+ "stations": "Hambly Beacon"
+ },
+ {
+ "name": "Lyncis Sector QJ-Q b5-2",
+ "pos_x": -99.75,
+ "pos_y": 100.53125,
+ "pos_z": -99.21875,
+ "stations": "The Blood of Atonement"
+ },
+ {
+ "name": "Lyncis Sector RO-R b4-5",
+ "pos_x": -123.28125,
+ "pos_y": 35.84375,
+ "pos_z": -121.9375,
+ "stations": "Caidin Base"
+ },
+ {
+ "name": "Lyncis Sector RT-R b4-1",
+ "pos_x": -83.03125,
+ "pos_y": 74.15625,
+ "pos_z": -123.15625,
+ "stations": "Hoffman Beacon"
+ },
+ {
+ "name": "Lyncis Sector TO-R b4-1",
+ "pos_x": -72.65625,
+ "pos_y": 46.21875,
+ "pos_z": -111.375,
+ "stations": "Andrews Observatory,Barton Bastion"
+ },
+ {
+ "name": "Lyncis Sector UZ-P b5-4",
+ "pos_x": -91.8125,
+ "pos_y": 66.96875,
+ "pos_z": -98.03125,
+ "stations": "Popper Refinery"
+ },
+ {
+ "name": "Lyncis Sector XJ-R b4-3",
+ "pos_x": -36.03125,
+ "pos_y": 25.5625,
+ "pos_z": -118.65625,
+ "stations": "Ferguson Settlement"
+ },
+ {
+ "name": "LZ Ursae Majoris",
+ "pos_x": -17.875,
+ "pos_y": 70.9375,
+ "pos_z": -42.375,
+ "stations": "Christopher Survey,Noether Dock"
+ },
+ {
+ "name": "M Hydrae",
+ "pos_x": 37.875,
+ "pos_y": 49.5625,
+ "pos_z": 76.5625,
+ "stations": "Maxwell Dock,Mawson Park,Acton Ring"
+ },
+ {
+ "name": "m Tauri",
+ "pos_x": 3.5625,
+ "pos_y": -10.78125,
+ "pos_z": -49.0625,
+ "stations": "Garay City,Wedge Station,Kooi Landing,Narvaez Vision,Birkeland Hub"
+ },
+ {
+ "name": "Ma'a",
+ "pos_x": -103.8125,
+ "pos_y": 55.0625,
+ "pos_z": -131.40625,
+ "stations": "Greenland Landing"
+ },
+ {
+ "name": "Ma'apocas",
+ "pos_x": -71.40625,
+ "pos_y": -53.25,
+ "pos_z": -99.125,
+ "stations": "Morgan City,Hewish Hub,Reilly Port,Buckland Refinery,Shepherd Plant"
+ },
+ {
+ "name": "Maasates",
+ "pos_x": 168.09375,
+ "pos_y": -148.25,
+ "pos_z": -33.875,
+ "stations": "Hay Hub,Pryor Legacy"
+ },
+ {
+ "name": "Maausk",
+ "pos_x": 98.40625,
+ "pos_y": -119.875,
+ "pos_z": 23.09375,
+ "stations": "Relph Orbital,Maausk,Seki Point,Emshwiller Depot"
+ },
+ {
+ "name": "Mabe",
+ "pos_x": -5.0625,
+ "pos_y": 46.28125,
+ "pos_z": 62.1875,
+ "stations": "Cummings Port,McMullen Port,Lehtonen Horizons,Agnesi Base,Williams Terminal"
+ },
+ {
+ "name": "Mabon",
+ "pos_x": 100.34375,
+ "pos_y": -160.8125,
+ "pos_z": -44.5625,
+ "stations": "Jewitt Hub,Lemonnier Orbital,Uto Orbital,Ocampo's Inheritance,Nearchus Enterprise"
+ },
+ {
+ "name": "Mabozho",
+ "pos_x": 139.75,
+ "pos_y": -178.5625,
+ "pos_z": 70.875,
+ "stations": "Kobayashi Retreat,Stone's Exile"
+ },
+ {
+ "name": "Mac Cha",
+ "pos_x": -24.25,
+ "pos_y": -161.03125,
+ "pos_z": -30.4375,
+ "stations": "Watanabe Dock,Vlaicu Dock,Zebrowski Town,Gorey Terminal"
+ },
+ {
+ "name": "Mac Cimi",
+ "pos_x": 37.84375,
+ "pos_y": -21.59375,
+ "pos_z": -124.90625,
+ "stations": "Shaver Terminal,Robinson City,Kazantsev Orbital,Janes Horizons,Aikin Dock,Foden Dock,Avogadro Holdings,Wilder Gateway,Speke Penal colony,Levi-Civita Camp,Carstensz Gateway,Perga Hub"
+ },
+ {
+ "name": "Mac Mari",
+ "pos_x": -151.65625,
+ "pos_y": -66.15625,
+ "pos_z": -89.9375,
+ "stations": "Tepper Port,Barr Beacon"
+ },
+ {
+ "name": "Mac Moti",
+ "pos_x": 181.8125,
+ "pos_y": -116.3125,
+ "pos_z": 45.5625,
+ "stations": "Picacio Settlement,Tall Hub"
+ },
+ {
+ "name": "Mac Og",
+ "pos_x": 80.9375,
+ "pos_y": -24.15625,
+ "pos_z": 84.90625,
+ "stations": "Amis Dock,Harness Dock"
+ },
+ {
+ "name": "Maca",
+ "pos_x": 98,
+ "pos_y": 16.375,
+ "pos_z": 93.875,
+ "stations": "Mendez Hub,Korniyenko Horizons,Laveykin Laboratory"
+ },
+ {
+ "name": "Macabi",
+ "pos_x": 109.8125,
+ "pos_y": 27.4375,
+ "pos_z": -2.125,
+ "stations": "Read Orbital,Russell Bastion,Aleksandrov Orbital,Hermaszewski Dock,Kekule Camp"
+ },
+ {
+ "name": "Macabika",
+ "pos_x": 124.09375,
+ "pos_y": -102.5625,
+ "pos_z": 95.09375,
+ "stations": "Takamizawa Arena"
+ },
+ {
+ "name": "Macabs",
+ "pos_x": 102.75,
+ "pos_y": 3.59375,
+ "pos_z": -14.59375,
+ "stations": "Midgley Dock,Chargaff Port,Boswell Penal colony"
+ },
+ {
+ "name": "Macalites",
+ "pos_x": 31.53125,
+ "pos_y": -18.21875,
+ "pos_z": 0.46875,
+ "stations": "Usachov City,Beckman Port,Banks Enterprise"
+ },
+ {
+ "name": "Macame",
+ "pos_x": 40.03125,
+ "pos_y": -63.9375,
+ "pos_z": 59.0625,
+ "stations": "Bauschinger Dock,Flettner Port"
+ },
+ {
+ "name": "Macap",
+ "pos_x": 127.28125,
+ "pos_y": -84.125,
+ "pos_z": 67.15625,
+ "stations": "Smirnova Works"
+ },
+ {
+ "name": "Macat",
+ "pos_x": 19.03125,
+ "pos_y": -145.125,
+ "pos_z": -24.53125,
+ "stations": "Taylor Jr. Station,Phillpotts Relay,Andree Base,Horrocks Dock"
+ },
+ {
+ "name": "Macay",
+ "pos_x": 127,
+ "pos_y": -111.09375,
+ "pos_z": -59.3125,
+ "stations": "Nijland Terminal,Pinzon Hub"
+ },
+ {
+ "name": "Mach",
+ "pos_x": -102.28125,
+ "pos_y": 58.59375,
+ "pos_z": 22.875,
+ "stations": "Henricks Outpost,Garriott Mine"
+ },
+ {
+ "name": "Machadan",
+ "pos_x": -158.34375,
+ "pos_y": 12.46875,
+ "pos_z": -39.90625,
+ "stations": "Bernoulli City,Galois Vision"
+ },
+ {
+ "name": "Machaquiaba",
+ "pos_x": 137.96875,
+ "pos_y": -163.28125,
+ "pos_z": 111.96875,
+ "stations": "Kapteyn Mines,Pannekoek Barracks,Helin Horizons"
+ },
+ {
+ "name": "Machatkwa",
+ "pos_x": 56.9375,
+ "pos_y": -138.875,
+ "pos_z": -63.8125,
+ "stations": "Ikeya Orbital,Alexander Ring,Zebrowski Barracks,Skjellerup Landing,Hay Installation"
+ },
+ {
+ "name": "Machi",
+ "pos_x": -19.40625,
+ "pos_y": 119.1875,
+ "pos_z": 7.3125,
+ "stations": "Evans Plant"
+ },
+ {
+ "name": "Machigga",
+ "pos_x": -73.3125,
+ "pos_y": 57.34375,
+ "pos_z": 97.25,
+ "stations": "Gooch Port,Bertin Settlement,Gernhardt Enterprise,Archimedes Hub,Sylvester's Inheritance"
+ },
+ {
+ "name": "Machimi",
+ "pos_x": 18.28125,
+ "pos_y": -234.875,
+ "pos_z": 67.09375,
+ "stations": "Skiff Relay"
+ },
+ {
+ "name": "Machola",
+ "pos_x": 125.75,
+ "pos_y": -61.375,
+ "pos_z": -66.96875,
+ "stations": "Mies van der Rohe Vision,Sheepshanks Landing,Baliunas Estate,Shklovsky Enterprise"
+ },
+ {
+ "name": "Macoc",
+ "pos_x": 85.15625,
+ "pos_y": -72,
+ "pos_z": 15.375,
+ "stations": "Marsden Plant,Barnard Enterprise"
+ },
+ {
+ "name": "Macoceleuta",
+ "pos_x": 61.25,
+ "pos_y": 2.59375,
+ "pos_z": -54.84375,
+ "stations": "Watson Hub,Hadfield Ring,Brothers Survey,Morin Station,Blodgett Installation"
+ },
+ {
+ "name": "Macrath",
+ "pos_x": -9545.15625,
+ "pos_y": -909.9375,
+ "pos_z": 19818.96875,
+ "stations": "Spassky's Prospect"
+ },
+ {
+ "name": "Macu Kop",
+ "pos_x": -54.59375,
+ "pos_y": -115.1875,
+ "pos_z": 118.75,
+ "stations": "Clute Hub,Shawl Prospect,Norgay Plant"
+ },
+ {
+ "name": "Macu Ku",
+ "pos_x": 145.78125,
+ "pos_y": -124.875,
+ "pos_z": -41.6875,
+ "stations": "Leberecht Tempel's Claim"
+ },
+ {
+ "name": "Macu Kuang",
+ "pos_x": 21.59375,
+ "pos_y": -88.34375,
+ "pos_z": 8.75,
+ "stations": "Sarafanov Settlement,Linnehan Works,Gardner Arsenal"
+ },
+ {
+ "name": "Macu Kung",
+ "pos_x": -36.875,
+ "pos_y": 77.59375,
+ "pos_z": 43.78125,
+ "stations": "Taine Colony,Tudela Installation"
+ },
+ {
+ "name": "Macua",
+ "pos_x": -46.03125,
+ "pos_y": -0.90625,
+ "pos_z": 160.53125,
+ "stations": "Brillant Relay,Piccard Oasis"
+ },
+ {
+ "name": "Macuano",
+ "pos_x": -39.34375,
+ "pos_y": -136.40625,
+ "pos_z": 56.03125,
+ "stations": "Parker Colony"
+ },
+ {
+ "name": "Macuayan",
+ "pos_x": 51.625,
+ "pos_y": -101.1875,
+ "pos_z": 101.34375,
+ "stations": "Tarter Dock,Laurent Relay,Farghani Orbital"
+ },
+ {
+ "name": "Macushi",
+ "pos_x": -93.5625,
+ "pos_y": -22.90625,
+ "pos_z": -115.4375,
+ "stations": "Georg Bothe Dock,Lorentz Dock,McArthur Orbital,Marusek Base"
+ },
+ {
+ "name": "Madimo",
+ "pos_x": -73.96875,
+ "pos_y": -85.71875,
+ "pos_z": 56.6875,
+ "stations": "Sinisalo Terminal,Ricci Landing,Sabine Holdings"
+ },
+ {
+ "name": "Madjandji",
+ "pos_x": -157,
+ "pos_y": -38.40625,
+ "pos_z": 16,
+ "stations": "Weitz Orbital"
+ },
+ {
+ "name": "Madngela",
+ "pos_x": 82.5625,
+ "pos_y": -197.3125,
+ "pos_z": 31.875,
+ "stations": "Merril Terminal,Bierce Station,Napier Dock,Barnes Hub"
+ },
+ {
+ "name": "Madngenses",
+ "pos_x": 155.46875,
+ "pos_y": -204.40625,
+ "pos_z": 16.34375,
+ "stations": "Luu Retreat,Kludze Port,Slade Hub"
+ },
+ {
+ "name": "Madngeri",
+ "pos_x": -36.71875,
+ "pos_y": 10.9375,
+ "pos_z": -93.25,
+ "stations": "Lunan Horizons,Phillips City,Evans Dock,Kuttner Terminal,Poncelet Enterprise,Garfinkle Hub,Kratman Orbital,Janifer Enterprise,Burkin Point,Maclaurin Enterprise"
+ },
+ {
+ "name": "Madngernobo",
+ "pos_x": -91.1875,
+ "pos_y": -5.15625,
+ "pos_z": -108.625,
+ "stations": "Delbruck Port,Junlong Hub,Fraley Ring"
+ },
+ {
+ "name": "Mado",
+ "pos_x": 110.375,
+ "pos_y": 32.5625,
+ "pos_z": 26.59375,
+ "stations": "Burnham Dock,Sohl Landing,Bushkov Station"
+ },
+ {
+ "name": "Madon",
+ "pos_x": -38.875,
+ "pos_y": -61.9375,
+ "pos_z": 43.375,
+ "stations": "Huberath Gateway,Schlegel Enterprise"
+ },
+ {
+ "name": "Madri",
+ "pos_x": -0.59375,
+ "pos_y": -177.125,
+ "pos_z": -13.25,
+ "stations": "Firsoff Colony,Russell Hub,Miyasaka Station"
+ },
+ {
+ "name": "Madrus",
+ "pos_x": -91.84375,
+ "pos_y": 76.34375,
+ "pos_z": 59.21875,
+ "stations": "Banach Settlement,Ordway Base"
+ },
+ {
+ "name": "Madutja",
+ "pos_x": -62.5,
+ "pos_y": -3.96875,
+ "pos_z": -163.3125,
+ "stations": "Sharp Station,Jones Beacon,Rae Dock,Navigator Station"
+ },
+ {
+ "name": "Madyaksha",
+ "pos_x": 89.625,
+ "pos_y": -240.84375,
+ "pos_z": 55.75,
+ "stations": "Burnham Landing"
+ },
+ {
+ "name": "Madyanitou",
+ "pos_x": 59.375,
+ "pos_y": 41.59375,
+ "pos_z": -103.6875,
+ "stations": "Beregovoi Dock,Ray Silo"
+ },
+ {
+ "name": "Madyanmana",
+ "pos_x": -89.1875,
+ "pos_y": -6.59375,
+ "pos_z": 92.53125,
+ "stations": "Doi Terminal,Avicenna Enterprise,White Vision,Kondakova Hub,Malpighi Port,Conway Dock,Whitney Port,Barjavel Terminal,Cavalieri Silo,Rasch Enterprise,McMonagle's Pride,Bresnik Terminal"
+ },
+ {
+ "name": "Maeve",
+ "pos_x": -0.625,
+ "pos_y": -56.8125,
+ "pos_z": 82.4375,
+ "stations": "Akers Stop,Przhevalsky Base"
+ },
+ {
+ "name": "Maevelite",
+ "pos_x": -71.25,
+ "pos_y": -97.59375,
+ "pos_z": -14.09375,
+ "stations": "Burnet Orbital"
+ },
+ {
+ "name": "Maevella",
+ "pos_x": 30.3125,
+ "pos_y": -225.21875,
+ "pos_z": 30.21875,
+ "stations": "Somerville Survey,MacGill Beacon,Benford Arsenal"
+ },
+ {
+ "name": "Mafdeta",
+ "pos_x": -133.5,
+ "pos_y": -1.34375,
+ "pos_z": -94.03125,
+ "stations": "Turzillo Dock,Galindo Relay,Stjepan Seljan Installation"
+ },
+ {
+ "name": "Mafdetela",
+ "pos_x": -1.34375,
+ "pos_y": 101.25,
+ "pos_z": 43.6875,
+ "stations": "Vinge Beacon,Wallin Settlement"
+ },
+ {
+ "name": "Mafthrasika",
+ "pos_x": -36.34375,
+ "pos_y": -78.15625,
+ "pos_z": 150.9375,
+ "stations": "Gaspar de Portola Orbital"
+ },
+ {
+ "name": "Mafthrudii",
+ "pos_x": 81.6875,
+ "pos_y": -60.1875,
+ "pos_z": -50.90625,
+ "stations": "Shavyrin Station,Roed Odegaard Installation"
+ },
+ {
+ "name": "Mafthruthin",
+ "pos_x": -16.8125,
+ "pos_y": 72.25,
+ "pos_z": 108.65625,
+ "stations": "Zewail Terminal,Adamson Terminal,Thompson Terminal,Gaffney Orbital,Bulleid Terminal,Toll Prospect,Samuda Horizons"
+ },
+ {
+ "name": "Magaitaka",
+ "pos_x": 118.59375,
+ "pos_y": -20.40625,
+ "pos_z": 108.125,
+ "stations": "Robson Orbital,Sekelj Plant"
+ },
+ {
+ "name": "Magali",
+ "pos_x": -76.34375,
+ "pos_y": 66.90625,
+ "pos_z": -86.0625,
+ "stations": "Fremont Market,Strzelecki Enterprise,Asher Dock,Evans Survey,Carver Port,Bruce Hub,Greenland Prospect"
+ },
+ {
+ "name": "Magari",
+ "pos_x": 1.46875,
+ "pos_y": 70.90625,
+ "pos_z": 137.59375,
+ "stations": "Baturin Station,Sharman Station,Reilly Hub"
+ },
+ {
+ "name": "Magec",
+ "pos_x": -32.875,
+ "pos_y": 36.15625,
+ "pos_z": 15.5,
+ "stations": "Arrhenius Colony,Good Keep,Trumpler Dock,Xiaoguan Hub,Schmitz Reach"
+ },
+ {
+ "name": "Magellan",
+ "pos_x": -9509.3125,
+ "pos_y": -914.625,
+ "pos_z": 19819.96875,
+ "stations": "Walhalla Port"
+ },
+ {
+ "name": "Maghiri",
+ "pos_x": 67.09375,
+ "pos_y": -19.21875,
+ "pos_z": -12.40625,
+ "stations": "Garnier Station,Coelho Barracks,Pierce Beacon"
+ },
+ {
+ "name": "Maghirvii",
+ "pos_x": -1,
+ "pos_y": 43.75,
+ "pos_z": -68.65625,
+ "stations": "Grothendieck Enterprise,Magnus Station"
+ },
+ {
+ "name": "Maghri",
+ "pos_x": 64.8125,
+ "pos_y": 117.84375,
+ "pos_z": -16.875,
+ "stations": "Meikle Orbital"
+ },
+ {
+ "name": "Magn",
+ "pos_x": 101.375,
+ "pos_y": -64.09375,
+ "pos_z": 78.21875,
+ "stations": "Brorsen Port,Burnham Hub"
+ },
+ {
+ "name": "Mahadshu",
+ "pos_x": -80.84375,
+ "pos_y": 5.625,
+ "pos_z": 105.6875,
+ "stations": "Peterson Base,Filipchenko Dock,Aristotle Landing"
+ },
+ {
+ "name": "Mahaes",
+ "pos_x": -102.59375,
+ "pos_y": 122.09375,
+ "pos_z": 50.21875,
+ "stations": "Treshchov Settlement,Stein Base,Sinclair Installation,McKay Landing"
+ },
+ {
+ "name": "Mahamanpa",
+ "pos_x": -43.8125,
+ "pos_y": 42.0625,
+ "pos_z": 122.46875,
+ "stations": "Vizcaino Enterprise,Shull Keep"
+ },
+ {
+ "name": "Mahar",
+ "pos_x": 118.5625,
+ "pos_y": -141.03125,
+ "pos_z": -20.375,
+ "stations": "Havilland Mine"
+ },
+ {
+ "name": "Mahara",
+ "pos_x": -67.59375,
+ "pos_y": 116.15625,
+ "pos_z": -40.75,
+ "stations": "Gallun City,Shiras Keep,Cummings Prospect"
+ },
+ {
+ "name": "Mahari",
+ "pos_x": 58.78125,
+ "pos_y": 13.125,
+ "pos_z": -80.65625,
+ "stations": "Nobel Gateway,al-Khayyam Orbital,Baker Observatory,Altshuller Arsenal,Popper Laboratory"
+ },
+ {
+ "name": "Mahatrents",
+ "pos_x": -149.3125,
+ "pos_y": 81.78125,
+ "pos_z": -51.40625,
+ "stations": "Schrodinger Works"
+ },
+ {
+ "name": "Mahau",
+ "pos_x": 118.34375,
+ "pos_y": -88.875,
+ "pos_z": 83.15625,
+ "stations": "Bering Settlement,Juan de la Cierva Port,Trujillo Mines"
+ },
+ {
+ "name": "Mahei",
+ "pos_x": 41.21875,
+ "pos_y": -97.96875,
+ "pos_z": -0.59375,
+ "stations": "Kempf Refinery"
+ },
+ {
+ "name": "Maheim",
+ "pos_x": 113.78125,
+ "pos_y": -27.84375,
+ "pos_z": 49.03125,
+ "stations": "Babbage Station,Schiltberger Keep"
+ },
+ {
+ "name": "Mahene",
+ "pos_x": -20.375,
+ "pos_y": -140.28125,
+ "pos_z": -32.625,
+ "stations": "Brooks Beacon,Levchenko Settlement"
+ },
+ {
+ "name": "Maheo",
+ "pos_x": 46.21875,
+ "pos_y": 74.6875,
+ "pos_z": -65.21875,
+ "stations": "Leestma Terminal"
+ },
+ {
+ "name": "Maheon",
+ "pos_x": 129.9375,
+ "pos_y": -142.5,
+ "pos_z": -32.5,
+ "stations": "Vavrova Port,Alvares Barracks,Roskam's Claim"
+ },
+ {
+ "name": "Maheou Ti",
+ "pos_x": -27.65625,
+ "pos_y": 35.25,
+ "pos_z": 73.09375,
+ "stations": "Feynman Station,Merbold Port,Ray City,Wang Enterprise,Yegorov Station,Bierce Beacon"
+ },
+ {
+ "name": "Mahia",
+ "pos_x": 77.9375,
+ "pos_y": -192.65625,
+ "pos_z": 104.75,
+ "stations": "Murakami Vision,Houten-Groeneveld Station,Gehry Station,Marsden's Inheritance"
+ },
+ {
+ "name": "Mahibitou",
+ "pos_x": 100.5625,
+ "pos_y": -58.5625,
+ "pos_z": -34.0625,
+ "stations": "Rucker Prospect,Duke Landing,Stafford Hub"
+ },
+ {
+ "name": "Mahiko",
+ "pos_x": 103.0625,
+ "pos_y": 1.5625,
+ "pos_z": 16.4375,
+ "stations": "Hertz Colony,Bartlett Survey"
+ },
+ {
+ "name": "Mahimata",
+ "pos_x": 47.65625,
+ "pos_y": -42.3125,
+ "pos_z": 36.125,
+ "stations": "White City,Glidden Penal colony,Burnell Enterprise"
+ },
+ {
+ "name": "Mahin",
+ "pos_x": 135.53125,
+ "pos_y": -141.9375,
+ "pos_z": 104.6875,
+ "stations": "Hughes' Folly,Hamuy Terminal,Sei Hub"
+ },
+ {
+ "name": "Mahina",
+ "pos_x": 142.3125,
+ "pos_y": -121.34375,
+ "pos_z": 57.34375,
+ "stations": "Thierree Hub,Leberecht Tempel Vision,Mattei Landing"
+ },
+ {
+ "name": "Mahlanja",
+ "pos_x": 121.15625,
+ "pos_y": 31.1875,
+ "pos_z": -37.3125,
+ "stations": "Vetulani Dock,Vinci City,Dobrovolski Orbital,Polyakov Ring,Payette Port,Clifton Depot,Readdy Gateway,Kaleri Gateway,Barjavel Landing,Drake Works,White Dock,Darwin Enterprise"
+ },
+ {
+ "name": "Mahlina",
+ "pos_x": 142.09375,
+ "pos_y": 29.3125,
+ "pos_z": -100.59375,
+ "stations": "Converse Legacy"
+ },
+ {
+ "name": "Mahomawi",
+ "pos_x": -22.71875,
+ "pos_y": 38.4375,
+ "pos_z": -101.59375,
+ "stations": "Banks Depot"
+ },
+ {
+ "name": "Maia",
+ "pos_x": -81.78125,
+ "pos_y": -149.4375,
+ "pos_z": -343.375,
+ "stations": "Obsidian Orbital,Darnielle's Progress,Palin Research Centre,Maia Point,Moni's Hub,Rescue Ship - Obsidian Orbital"
+ },
+ {
+ "name": "Maiadiwobog",
+ "pos_x": 144.3125,
+ "pos_y": -180.9375,
+ "pos_z": -53.96875,
+ "stations": "Bullialdus Prospect,Elmore Landing,van de Sande Bakhuyzen Depot"
+ },
+ {
+ "name": "Maiawa",
+ "pos_x": 23.21875,
+ "pos_y": 49.8125,
+ "pos_z": -66.6875,
+ "stations": "Clement Landing,Reed Platform,Sekelj Settlement"
+ },
+ {
+ "name": "Maid Ollanque",
+ "pos_x": -19.5,
+ "pos_y": -77.71875,
+ "pos_z": 90.625,
+ "stations": "Sabine Freeport,Yourkevitch Platform"
+ },
+ {
+ "name": "Maidareldi",
+ "pos_x": -40.78125,
+ "pos_y": -21.53125,
+ "pos_z": -140.625,
+ "stations": "Cogswell Depot,Grandin Beacon,Wisoff Hangar"
+ },
+ {
+ "name": "Maiden",
+ "pos_x": -8,
+ "pos_y": -98.28125,
+ "pos_z": -51.40625,
+ "stations": "Leberecht Tempel Orbital,Hoerner Dock,Bruce's Folly,Baxter's Folly,George's Inheritance,Heinkel Terminal,Dyr Terminal,Kawanishi Station,Nicolet Installation"
+ },
+ {
+ "name": "Maidenwites",
+ "pos_x": 160.15625,
+ "pos_y": -101.21875,
+ "pos_z": 56.65625,
+ "stations": "Roche Settlement,Israel Terminal,Mandel Holdings,Haro Vision"
+ },
+ {
+ "name": "Maidjigak",
+ "pos_x": -16.1875,
+ "pos_y": -98.875,
+ "pos_z": 35.71875,
+ "stations": "Cunningham Refinery,Cassidy City"
+ },
+ {
+ "name": "Maidjin",
+ "pos_x": 33.15625,
+ "pos_y": -85.75,
+ "pos_z": 99.71875,
+ "stations": "Clebsch Terminal,Abetti Survey,Filippenko Enterprise,Shoujing Laboratory"
+ },
+ {
+ "name": "Maidubii",
+ "pos_x": -105.90625,
+ "pos_y": 2.71875,
+ "pos_z": -49.65625,
+ "stations": "Nielsen Orbital,Landsteiner Penal colony,Wul Laboratory"
+ },
+ {
+ "name": "Maidubrigel",
+ "pos_x": 82.03125,
+ "pos_y": -142.09375,
+ "pos_z": 51.1875,
+ "stations": "MacGill Estate,Barmin City"
+ },
+ {
+ "name": "Maiducane",
+ "pos_x": 44.34375,
+ "pos_y": -76.0625,
+ "pos_z": 89.125,
+ "stations": "Juan de la Cierva Hub,Cerulli Landing"
+ },
+ {
+ "name": "Maijarua",
+ "pos_x": -13.40625,
+ "pos_y": 7.46875,
+ "pos_z": 78.46875,
+ "stations": "Usachov Point,Sellers Gateway,Nagel City,Musgrave Dock"
+ },
+ {
+ "name": "Maijuhaca",
+ "pos_x": -91.25,
+ "pos_y": 160.8125,
+ "pos_z": 53.53125,
+ "stations": "Binder Point,Henslow Depot"
+ },
+ {
+ "name": "Maik",
+ "pos_x": -10.46875,
+ "pos_y": -1.0625,
+ "pos_z": 101.28125,
+ "stations": "Womack Hub,Howard Point"
+ },
+ {
+ "name": "Maiki",
+ "pos_x": 98.3125,
+ "pos_y": -170.84375,
+ "pos_z": 89.5,
+ "stations": "Zenbei Port,Neumann Relay,Weizsacker Terminal,Lagerkvist Vision,Salpeter Terminal"
+ },
+ {
+ "name": "Maikoro",
+ "pos_x": -75.8125,
+ "pos_y": -19.46875,
+ "pos_z": -27.84375,
+ "stations": "Hawking Port,Kummer Depot"
+ },
+ {
+ "name": "Maikpod",
+ "pos_x": 52.375,
+ "pos_y": -227.0625,
+ "pos_z": 88.3125,
+ "stations": "Pritchard Orbital,Ludwig Struve Prospect,Bisnovatyi-Kogan Landing,Henson Town"
+ },
+ {
+ "name": "Maiku",
+ "pos_x": 95.03125,
+ "pos_y": -81.65625,
+ "pos_z": -39.40625,
+ "stations": "Austin Station,Thomas Holdings,Tsiolkovsky Dock,Mueller Gateway,Lee Point"
+ },
+ {
+ "name": "Main",
+ "pos_x": -22.34375,
+ "pos_y": -80.71875,
+ "pos_z": 39.625,
+ "stations": "Zhigang Point,Virts' Inheritance"
+ },
+ {
+ "name": "Mainani",
+ "pos_x": 10.40625,
+ "pos_y": -219.8125,
+ "pos_z": -64.21875,
+ "stations": "Mies van der Rohe's Claim"
+ },
+ {
+ "name": "Maipaera",
+ "pos_x": -129.21875,
+ "pos_y": 4.90625,
+ "pos_z": -104.46875,
+ "stations": "Langsdorff Landing,Thurston Plant,Dantec Retreat"
+ },
+ {
+ "name": "Maiphu",
+ "pos_x": -37.125,
+ "pos_y": -37.25,
+ "pos_z": 163.78125,
+ "stations": "Wetherbee Vision"
+ },
+ {
+ "name": "Maipuna",
+ "pos_x": 66.6875,
+ "pos_y": -68.96875,
+ "pos_z": 117.3125,
+ "stations": "Carpenter Station,Barth Hub,Qureshi Ring"
+ },
+ {
+ "name": "Maitha",
+ "pos_x": 40.09375,
+ "pos_y": 6,
+ "pos_z": 127.21875,
+ "stations": "Zamka Port"
+ },
+ {
+ "name": "Maitis",
+ "pos_x": -84.1875,
+ "pos_y": 18.0625,
+ "pos_z": -47.28125,
+ "stations": "Searfoss Refinery,Pavlov Beacon,Parsons City"
+ },
+ {
+ "name": "Maityan",
+ "pos_x": 85.46875,
+ "pos_y": -78.46875,
+ "pos_z": 59.28125,
+ "stations": "Maughmer Ring,Sunyaev Observatory,Fabian Enterprise,Pickering Enterprise"
+ },
+ {
+ "name": "Maityati",
+ "pos_x": -92.09375,
+ "pos_y": 40,
+ "pos_z": 90.0625,
+ "stations": "Morey Gateway"
+ },
+ {
+ "name": "Majhia",
+ "pos_x": -5.03125,
+ "pos_y": -210.125,
+ "pos_z": 46.84375,
+ "stations": "Dyr Station,Velho Terminal,Shaw Prospect,Gabrielli's Inheritance"
+ },
+ {
+ "name": "Majhiat",
+ "pos_x": -114.375,
+ "pos_y": -39.5,
+ "pos_z": -56.59375,
+ "stations": "Lonchakov Station,Still Station,Tombaugh Enterprise,Harper Settlement,Plucker Settlement"
+ },
+ {
+ "name": "Majhwala",
+ "pos_x": 0.78125,
+ "pos_y": -122.5,
+ "pos_z": -27.40625,
+ "stations": "Chawla Settlement,Ingstad Vision,Stein Dock,Shoemaker Vision"
+ },
+ {
+ "name": "Majhwar",
+ "pos_x": -41.5625,
+ "pos_y": -46.40625,
+ "pos_z": 98.78125,
+ "stations": "Antonio de Andrade Station,Bond City,Cooper City,Tavernier Terminal,Arthur Orbital,Mallett Depot,Mitchell Depot,Yourkevitch Beacon"
+ },
+ {
+ "name": "Maka",
+ "pos_x": 84.90625,
+ "pos_y": -19.8125,
+ "pos_z": 22.28125,
+ "stations": "Battuta Beacon,Winne Outpost"
+ },
+ {
+ "name": "Makah",
+ "pos_x": 15.65625,
+ "pos_y": -42.5625,
+ "pos_z": -94.8125,
+ "stations": "Jacquard Works"
+ },
+ {
+ "name": "Makaneane",
+ "pos_x": 51.34375,
+ "pos_y": 37.3125,
+ "pos_z": -62.1875,
+ "stations": "Hawkes Point,Maclaurin Dock,Henize Horizons,Clairaut Installation"
+ },
+ {
+ "name": "Makasing Yi",
+ "pos_x": 37.28125,
+ "pos_y": -59.5625,
+ "pos_z": -108.53125,
+ "stations": "Dyr Ring,Ingstad Ring,Gabriel Penal colony,Kroehl Observatory"
+ },
+ {
+ "name": "Makau",
+ "pos_x": -18.3125,
+ "pos_y": -158.46875,
+ "pos_z": 133.25,
+ "stations": "Turner Dock,Sagan Hub"
+ },
+ {
+ "name": "Maker",
+ "pos_x": 157.875,
+ "pos_y": -207.625,
+ "pos_z": 33.84375,
+ "stations": "Royo Terminal,Shimizu Vision,Barlowe Survey,Weiss Market"
+ },
+ {
+ "name": "Mako",
+ "pos_x": 100.5625,
+ "pos_y": -85.25,
+ "pos_z": 134.21875,
+ "stations": "Abell Dock,Truman Colony"
+ },
+ {
+ "name": "Makota",
+ "pos_x": 166.34375,
+ "pos_y": -123.8125,
+ "pos_z": 107.21875,
+ "stations": "Bohme Port,Lockyer Terminal,Asire Plant,Bloch Installation"
+ },
+ {
+ "name": "Makotamona",
+ "pos_x": -78.625,
+ "pos_y": -39.59375,
+ "pos_z": -120.15625,
+ "stations": "Kennicott Terminal,Alexandria Platform"
+ },
+ {
+ "name": "Makula",
+ "pos_x": -113.15625,
+ "pos_y": -19,
+ "pos_z": -96.625,
+ "stations": "Neumann Settlement,Xing Orbital,Kotov Platform"
+ },
+ {
+ "name": "Makulcaei",
+ "pos_x": 155.625,
+ "pos_y": -73.46875,
+ "pos_z": -87.5625,
+ "stations": "Fox Landing,Druillet Dock,Moore Installation"
+ },
+ {
+ "name": "Makulu",
+ "pos_x": -88.9375,
+ "pos_y": 56.46875,
+ "pos_z": 21.1875,
+ "stations": "Hubble City,Hahn Orbital,Scobee Terminal,Gibson Ring,Zholobov Orbital,Wegener Gateway,Hawking Terminal,Bentham Penal colony,Sekowski Laboratory,Moresby's Folly"
+ },
+ {
+ "name": "Makuluk",
+ "pos_x": 11.28125,
+ "pos_y": 70.53125,
+ "pos_z": 115.6875,
+ "stations": "Shatalov Dock,Oramus Horizons,Gresley Legacy"
+ },
+ {
+ "name": "Makum",
+ "pos_x": 18.4375,
+ "pos_y": 33,
+ "pos_z": -121.9375,
+ "stations": "Pytheas Terminal,Burgess Terminal,Poincare Enterprise,Anthony Prospect,Sagan Relay"
+ },
+ {
+ "name": "Makurboda",
+ "pos_x": 80.90625,
+ "pos_y": 38.21875,
+ "pos_z": 104.53125,
+ "stations": "Rushd Ring,Polansky Landing,Wheeler Enterprise,Grothendieck Vision,Cook Relay"
+ },
+ {
+ "name": "Mal Yarr",
+ "pos_x": 136.4375,
+ "pos_y": -130.90625,
+ "pos_z": 56.40625,
+ "stations": "Martiniere Vision,Grant Point"
+ },
+ {
+ "name": "Mal Ye",
+ "pos_x": 53,
+ "pos_y": -152.3125,
+ "pos_z": 7.5625,
+ "stations": "Sy Landing,Hall Port"
+ },
+ {
+ "name": "Malae",
+ "pos_x": -81.125,
+ "pos_y": 105.625,
+ "pos_z": 152.28125,
+ "stations": "de Balboa Beacon,Gann Port,Tarentum Depot"
+ },
+ {
+ "name": "Malaikudi",
+ "pos_x": 97.75,
+ "pos_y": -41.15625,
+ "pos_z": -51,
+ "stations": "Leopold Heckmann Ring,Kahn Escape,Schmidt Silo"
+ },
+ {
+ "name": "Malaji",
+ "pos_x": 127.3125,
+ "pos_y": -37.90625,
+ "pos_z": -75.625,
+ "stations": "Whitson Dock,Hart Station,Szameit Survey"
+ },
+ {
+ "name": "Malak Malak",
+ "pos_x": 193.65625,
+ "pos_y": -136.125,
+ "pos_z": 60.09375,
+ "stations": "Zelazny Barracks,Bohr Enterprise,Zel'dovich Hub"
+ },
+ {
+ "name": "Malaku",
+ "pos_x": -69.09375,
+ "pos_y": 109.375,
+ "pos_z": 42.3125,
+ "stations": "Yu Base,Grego Platform,Sarrantonio Settlement,Shalatula Lab"
+ },
+ {
+ "name": "Malali",
+ "pos_x": 27.3125,
+ "pos_y": 67.75,
+ "pos_z": 118.1875,
+ "stations": "Janifer Plant,McIntyre Refinery"
+ },
+ {
+ "name": "Malamal",
+ "pos_x": -2.125,
+ "pos_y": -121.15625,
+ "pos_z": 80.8125,
+ "stations": "Youll City"
+ },
+ {
+ "name": "Malaman",
+ "pos_x": -156.9375,
+ "pos_y": 33.9375,
+ "pos_z": 53.03125,
+ "stations": "Chomsky Colony,Adams Terminal"
+ },
+ {
+ "name": "Malameni",
+ "pos_x": -127.3125,
+ "pos_y": 89.4375,
+ "pos_z": -62.75,
+ "stations": "Webb Depot"
+ },
+ {
+ "name": "Malanges",
+ "pos_x": 22.53125,
+ "pos_y": -83.59375,
+ "pos_z": -154.65625,
+ "stations": "Heng Ring,Santos Terminal,Rashid Port,Rzeppa Orbital"
+ },
+ {
+ "name": "Malangga",
+ "pos_x": 45.71875,
+ "pos_y": -27.90625,
+ "pos_z": -68.46875,
+ "stations": "Lehtonen's Inheritance,Taylor Station,Bushkov Orbital,Priest Co-operative,Ron Hubbard Enterprise"
+ },
+ {
+ "name": "Malannher",
+ "pos_x": 41.625,
+ "pos_y": -69.90625,
+ "pos_z": -14.09375,
+ "stations": "Zelazny Vista,Readdy Park,Khan City,Brash Terminal,Lanchester Dock"
+ },
+ {
+ "name": "Malanquiya",
+ "pos_x": -150.21875,
+ "pos_y": 30.09375,
+ "pos_z": -15.6875,
+ "stations": "Stross Camp,Bertin Vision"
+ },
+ {
+ "name": "Malapa",
+ "pos_x": 22.9375,
+ "pos_y": -189.46875,
+ "pos_z": 15.96875,
+ "stations": "Wendelin Depot,Gunter Settlement"
+ },
+ {
+ "name": "Malarhones",
+ "pos_x": 80.65625,
+ "pos_y": -78.125,
+ "pos_z": 36.5625,
+ "stations": "Delsanti Ring,Kurtz Dock,Pickering Dock,Huggins Forum"
+ },
+ {
+ "name": "Malarni",
+ "pos_x": 17.03125,
+ "pos_y": -19.34375,
+ "pos_z": 139.78125,
+ "stations": "Mallory Prospect,Barnaby Outpost"
+ },
+ {
+ "name": "Malaroecavi",
+ "pos_x": 89.78125,
+ "pos_y": -123.84375,
+ "pos_z": 107.28125,
+ "stations": "Syromyatnikov Survey,Pilyugin Mines,Salpeter Depot,Seitter Base"
+ },
+ {
+ "name": "Malatia",
+ "pos_x": 34.875,
+ "pos_y": 97.53125,
+ "pos_z": 16.625,
+ "stations": "Pinto's Inheritance,Hirase Station,Gurragchaa Landing,Lind Beacon"
+ },
+ {
+ "name": "Malayamatha",
+ "pos_x": 113.4375,
+ "pos_y": 0.09375,
+ "pos_z": -45.71875,
+ "stations": "Gernhardt Orbital,Salk Terminal"
+ },
+ {
+ "name": "Malayat",
+ "pos_x": -85.0625,
+ "pos_y": -85.78125,
+ "pos_z": -123.15625,
+ "stations": "Schouten Stop"
+ },
+ {
+ "name": "Malean",
+ "pos_x": -37.90625,
+ "pos_y": 34.125,
+ "pos_z": -96.53125,
+ "stations": "Al Saud Gateway,Makarov Colony,Tenn Silo,Wang Dock,Pailes Landing"
+ },
+ {
+ "name": "Maleenens",
+ "pos_x": -40.6875,
+ "pos_y": -140.5625,
+ "pos_z": 37.4375,
+ "stations": "Chernykh Port,Makeev Barracks"
+ },
+ {
+ "name": "Maleku",
+ "pos_x": -132.59375,
+ "pos_y": -100.21875,
+ "pos_z": -40.40625,
+ "stations": "Payton Port"
+ },
+ {
+ "name": "Malga",
+ "pos_x": 37.90625,
+ "pos_y": -80.78125,
+ "pos_z": 103.46875,
+ "stations": "Carroll Port,Meaney Enterprise,McDermott Horizons"
+ },
+ {
+ "name": "Malgal",
+ "pos_x": -164.59375,
+ "pos_y": -32.375,
+ "pos_z": -33.6875,
+ "stations": "Tanner Beacon"
+ },
+ {
+ "name": "Malgar",
+ "pos_x": 102.78125,
+ "pos_y": -48.625,
+ "pos_z": 59.15625,
+ "stations": "Arisman Survey"
+ },
+ {
+ "name": "Malgariji",
+ "pos_x": 80.9375,
+ "pos_y": 16.28125,
+ "pos_z": -142.78125,
+ "stations": "Hire Colony,Macarthur Ring,Chretien Penal colony"
+ },
+ {
+ "name": "Mali",
+ "pos_x": 28.78125,
+ "pos_y": -20.59375,
+ "pos_z": 148.9375,
+ "stations": "Otiman Enterprise,Detmer Ring,Vancouver Installation"
+ },
+ {
+ "name": "Malibata",
+ "pos_x": 72.1875,
+ "pos_y": -54.375,
+ "pos_z": 83.90625,
+ "stations": "Rittenhouse Settlement,Lemonnier Hangar"
+ },
+ {
+ "name": "Malina",
+ "pos_x": -48.1875,
+ "pos_y": 23,
+ "pos_z": 14.40625,
+ "stations": "Kooi Resort"
+ },
+ {
+ "name": "Malja",
+ "pos_x": -46.125,
+ "pos_y": 65.03125,
+ "pos_z": 86.5,
+ "stations": "Griffith Survey"
+ },
+ {
+ "name": "Maljadal",
+ "pos_x": 66.1875,
+ "pos_y": 11.46875,
+ "pos_z": 126.21875,
+ "stations": "Rutherford Mines"
+ },
+ {
+ "name": "Maljan",
+ "pos_x": 72.625,
+ "pos_y": -0.875,
+ "pos_z": -127.84375,
+ "stations": "Musa al-Khwarizmi Platform"
+ },
+ {
+ "name": "Maljang",
+ "pos_x": -24.28125,
+ "pos_y": 74.8125,
+ "pos_z": 105.875,
+ "stations": "Frobisher Enterprise,Gunn Terminal,Viete Terminal,Chandler Orbital"
+ },
+ {
+ "name": "Maljangapa",
+ "pos_x": 142.09375,
+ "pos_y": -68.71875,
+ "pos_z": 78.78125,
+ "stations": "Allen St. John Relay,Furukawa Base,Argelander Landing"
+ },
+ {
+ "name": "Maljenni",
+ "pos_x": 61.34375,
+ "pos_y": 38.71875,
+ "pos_z": 17,
+ "stations": "Bowersox Enterprise,Satcher Hub,Drew Vision,Strekalov Enterprise"
+ },
+ {
+ "name": "Malloc",
+ "pos_x": -65.1875,
+ "pos_y": 5.71875,
+ "pos_z": 59.71875,
+ "stations": "Onizuka Ring,Julian Gateway,Morin Relay,Carter Base"
+ },
+ {
+ "name": "Malpar Paha",
+ "pos_x": -51.46875,
+ "pos_y": 52.625,
+ "pos_z": -80.21875,
+ "stations": "Ericsson Colony,Trinh Settlement,McKay's Folly"
+ },
+ {
+ "name": "Malparci",
+ "pos_x": -161.71875,
+ "pos_y": -21.90625,
+ "pos_z": -15.65625,
+ "stations": "Schmitz Point,Cook Settlement,Cabral Enterprise"
+ },
+ {
+ "name": "Malpi",
+ "pos_x": -144.78125,
+ "pos_y": 31.6875,
+ "pos_z": -15.5625,
+ "stations": "Volynov Port"
+ },
+ {
+ "name": "Mals",
+ "pos_x": -58.40625,
+ "pos_y": -79.25,
+ "pos_z": 88.96875,
+ "stations": "Murakami Dock"
+ },
+ {
+ "name": "Malsuman",
+ "pos_x": 106.84375,
+ "pos_y": -14.09375,
+ "pos_z": 25.34375,
+ "stations": "Rominger City,Bosch Station,Carver Gateway"
+ },
+ {
+ "name": "Malsungga",
+ "pos_x": -69.46875,
+ "pos_y": -76.6875,
+ "pos_z": 20.84375,
+ "stations": "Borlaug Port,McCoy Mines,Coulomb Beacon"
+ },
+ {
+ "name": "Malsunghus",
+ "pos_x": 125.375,
+ "pos_y": -215.75,
+ "pos_z": -6.46875,
+ "stations": "Perry Terminal,Mies van der Rohe Landing,Brom Prospect,Edwards Depot"
+ },
+ {
+ "name": "Mam",
+ "pos_x": 51.9375,
+ "pos_y": -23.25,
+ "pos_z": 97.0625,
+ "stations": "Taylor Reach,Frobenius Refinery"
+ },
+ {
+ "name": "Mamaragan",
+ "pos_x": 33.875,
+ "pos_y": -49.09375,
+ "pos_z": 26.4375,
+ "stations": "Padalka Orbital,Wolf Platform"
+ },
+ {
+ "name": "Mambaislas",
+ "pos_x": 14.96875,
+ "pos_y": 13.40625,
+ "pos_z": 102.15625,
+ "stations": "Glazkov Terminal,Kelly Beacon"
+ },
+ {
+ "name": "Mambo",
+ "pos_x": 34.5625,
+ "pos_y": 101.1875,
+ "pos_z": -130.625,
+ "stations": "Tesla Depot,Ising Survey"
+ },
+ {
+ "name": "Mambojas",
+ "pos_x": -8.875,
+ "pos_y": -16.09375,
+ "pos_z": 68.875,
+ "stations": "Patsayev Enterprise,Cowper Prospect,Conrad Station,Rennie Relay"
+ },
+ {
+ "name": "Mamitu",
+ "pos_x": 0.40625,
+ "pos_y": 40.625,
+ "pos_z": 40.84375,
+ "stations": "Bacigalupi Holdings,Steele Vision,Clervoy Plant"
+ },
+ {
+ "name": "Mammon",
+ "pos_x": -358.375,
+ "pos_y": -8.75,
+ "pos_z": 933.53125,
+ "stations": "Mammon Monitoring Facility"
+ },
+ {
+ "name": "Mamur",
+ "pos_x": 88.0625,
+ "pos_y": 30.03125,
+ "pos_z": -135.84375,
+ "stations": "Liwei Terminal"
+ },
+ {
+ "name": "Manabush",
+ "pos_x": -1.875,
+ "pos_y": 83.96875,
+ "pos_z": 135.375,
+ "stations": "Cavalieri Gateway,Dias Orbital"
+ },
+ {
+ "name": "Manah",
+ "pos_x": -6.96875,
+ "pos_y": -27.90625,
+ "pos_z": -50.125,
+ "stations": "Malchiodi Station,Petra Station"
+ },
+ {
+ "name": "Manahpuch",
+ "pos_x": -119.0625,
+ "pos_y": 82.9375,
+ "pos_z": 49.59375,
+ "stations": "Young Port,Ricci Settlement"
+ },
+ {
+ "name": "Manaku",
+ "pos_x": -17.15625,
+ "pos_y": -88.875,
+ "pos_z": 99.84375,
+ "stations": "Birdseye Landing,Drexler Orbital,Rasmussen Settlement"
+ },
+ {
+ "name": "Manamaya",
+ "pos_x": -29.40625,
+ "pos_y": 2.03125,
+ "pos_z": -61.21875,
+ "stations": "Cameron Survey,al-Haytham Enterprise,Shuttleworth Terminal"
+ },
+ {
+ "name": "Manapii",
+ "pos_x": 96.625,
+ "pos_y": 86.96875,
+ "pos_z": -7.125,
+ "stations": "Taylor's Inheritance,Bloch Dock,Stephens' Folly"
+ },
+ {
+ "name": "Manara",
+ "pos_x": -134.6875,
+ "pos_y": -60.375,
+ "pos_z": 107.65625,
+ "stations": "Hiroyuki Works"
+ },
+ {
+ "name": "Manarato",
+ "pos_x": -91.84375,
+ "pos_y": 64.9375,
+ "pos_z": -38.5,
+ "stations": "McIntyre Platform,Shelley Prospect,Macomb Dock"
+ },
+ {
+ "name": "Manatera",
+ "pos_x": 98.46875,
+ "pos_y": -85.46875,
+ "pos_z": -44.53125,
+ "stations": "Shajn Vision,Slusser Depot,Vaucouleurs Refinery,Acharya Beacon"
+ },
+ {
+ "name": "Manates",
+ "pos_x": 69.25,
+ "pos_y": 132.375,
+ "pos_z": -122.59375,
+ "stations": "May's Claim,Bryusov Mines"
+ },
+ {
+ "name": "Manathajeri",
+ "pos_x": -11.375,
+ "pos_y": 90.15625,
+ "pos_z": 69.8125,
+ "stations": "Lysenko Mine,Bennett Stop"
+ },
+ {
+ "name": "Manati",
+ "pos_x": -33.4375,
+ "pos_y": -159.0625,
+ "pos_z": 9.59375,
+ "stations": "Belyavsky Station,Gaughan Gateway,Skiff Hub,Abasheli Settlement"
+ },
+ {
+ "name": "Manawan",
+ "pos_x": 27.1875,
+ "pos_y": 76.84375,
+ "pos_z": 122.71875,
+ "stations": "Jolliet Hub,Chun Settlement,Zindell's Inheritance"
+ },
+ {
+ "name": "Manawydan",
+ "pos_x": -60.1875,
+ "pos_y": 160.96875,
+ "pos_z": -1.3125,
+ "stations": "Gottlob Frege Ring,Cook Park"
+ },
+ {
+ "name": "Manba",
+ "pos_x": 160.65625,
+ "pos_y": -163.53125,
+ "pos_z": -5.53125,
+ "stations": "Bliss Camp,Leberecht Tempel Survey,Gould Survey"
+ },
+ {
+ "name": "Manbatz",
+ "pos_x": -13.625,
+ "pos_y": -16.96875,
+ "pos_z": 95.5625,
+ "stations": "Bretnor Terminal,Herodotus Orbital,Balmer Gateway"
+ },
+ {
+ "name": "Manchi",
+ "pos_x": 171.90625,
+ "pos_y": -172.84375,
+ "pos_z": 17.1875,
+ "stations": "Hansen's Inheritance"
+ },
+ {
+ "name": "Mandal",
+ "pos_x": 81.375,
+ "pos_y": -49.5,
+ "pos_z": 79.0625,
+ "stations": "Chertovsky Landing,Anderson Point"
+ },
+ {
+ "name": "Mandelian",
+ "pos_x": 80.84375,
+ "pos_y": 35.375,
+ "pos_z": -93.46875,
+ "stations": "Weyn City,Caryanda Platform,Pytheas Camp"
+ },
+ {
+ "name": "Mandh",
+ "pos_x": 45.28125,
+ "pos_y": -98.53125,
+ "pos_z": -38.21875,
+ "stations": "Juan de la Cierva Horizons,Camm Vision,Banks Landing,Joseph Delambre Vision,Suntzeff Terminal,Miyasaka Orbital,Gaensler City,Ziegel Station,Oberth City"
+ },
+ {
+ "name": "Mandhata",
+ "pos_x": 57.6875,
+ "pos_y": -36.4375,
+ "pos_z": 143.4375,
+ "stations": "Gann Depot,Whitelaw Ring,Garden Works,Montrose Hub,Spinrad Terminal"
+ },
+ {
+ "name": "Mandhrithar",
+ "pos_x": 63.625,
+ "pos_y": -31.40625,
+ "pos_z": 67.28125,
+ "stations": "Phillifent Station,Amnuel City,Young Dock,O'Leary City,Siodmak City,Ziemianski City,Fox City,Dalmas Terminal,Sato Vision,Shen Colony,Dukaj Relay,Ferguson Hub"
+ },
+ {
+ "name": "Mandigo",
+ "pos_x": 139.5,
+ "pos_y": -133.125,
+ "pos_z": -55.21875,
+ "stations": "Muramatsu Station,Goodricke Horizons"
+ },
+ {
+ "name": "Mandii",
+ "pos_x": -92.59375,
+ "pos_y": -92.03125,
+ "pos_z": 46.4375,
+ "stations": "Haise Base,Garriott Mine,Ibold Settlement"
+ },
+ {
+ "name": "Mandill",
+ "pos_x": 77.5625,
+ "pos_y": -203.4375,
+ "pos_z": -64.5625,
+ "stations": "Cassini Terminal,Low Settlement,Fehrenbach Prospect"
+ },
+ {
+ "name": "Mandins",
+ "pos_x": 12.03125,
+ "pos_y": 78.8125,
+ "pos_z": 74.71875,
+ "stations": "Kaleri Camp,Weston Mine"
+ },
+ {
+ "name": "Mandjera",
+ "pos_x": -36.84375,
+ "pos_y": -41.9375,
+ "pos_z": -173.875,
+ "stations": "Penn Holdings,Taine Port,Panshin's Progress"
+ },
+ {
+ "name": "Mandjety",
+ "pos_x": 38.15625,
+ "pos_y": 41.15625,
+ "pos_z": 137.84375,
+ "stations": "Tryggvason Platform"
+ },
+ {
+ "name": "Mandjila",
+ "pos_x": 8,
+ "pos_y": -97.5625,
+ "pos_z": 43.40625,
+ "stations": "Crown Terminal,Irens Hub,King Enterprise,Blish Station,Oltion Station,Stephens Ring,Tarski Station,Levinson Depot,Elvstrom Landing,Gray Prospect"
+ },
+ {
+ "name": "Mandjindja",
+ "pos_x": -92.78125,
+ "pos_y": 106.5,
+ "pos_z": 109.9375,
+ "stations": "Bondar Station,McMonagle Dock,Hoshi's Pride,Dowling Installation"
+ },
+ {
+ "name": "Mandjirbole",
+ "pos_x": 26.46875,
+ "pos_y": -159.15625,
+ "pos_z": -79.1875,
+ "stations": "Wachmann Landing,Biruni Prospect"
+ },
+ {
+ "name": "Mandra",
+ "pos_x": 104.96875,
+ "pos_y": -117.40625,
+ "pos_z": -7.46875,
+ "stations": "Dunlop Terminal,Neujmin Port,Shklovsky Terminal"
+ },
+ {
+ "name": "Mandu",
+ "pos_x": 117.9375,
+ "pos_y": -22.1875,
+ "pos_z": 17.34375,
+ "stations": "Wang Prospect,Erdos Barracks"
+ },
+ {
+ "name": "Mandubii",
+ "pos_x": 116,
+ "pos_y": -32.125,
+ "pos_z": 99.75,
+ "stations": "Dyson Orbital,Meucci Landing"
+ },
+ {
+ "name": "Mang",
+ "pos_x": 21.6875,
+ "pos_y": -10.21875,
+ "pos_z": 88.3125,
+ "stations": "Pontes Terminal,Frobisher Installation,Reed Point"
+ },
+ {
+ "name": "Mangapa",
+ "pos_x": 1.09375,
+ "pos_y": 127.3125,
+ "pos_z": 53.71875,
+ "stations": "Savitskaya Landing,Berezin Settlement,Linnaeus Settlement"
+ },
+ {
+ "name": "Mangarai",
+ "pos_x": 118.25,
+ "pos_y": 59.1875,
+ "pos_z": -75.0625,
+ "stations": "Clapperton Dock,Kuipers Refinery,Whitelaw Works"
+ },
+ {
+ "name": "Mangiliri",
+ "pos_x": 76.71875,
+ "pos_y": 27.125,
+ "pos_z": -139.90625,
+ "stations": "Bailey Vision,Scortia Keep,Bunnell Prospect,Pytheas City"
+ },
+ {
+ "name": "Mangkhwal",
+ "pos_x": -73.15625,
+ "pos_y": -78.625,
+ "pos_z": -78.78125,
+ "stations": "Alten City,O'Leary Landing,Haldeman II Relay"
+ },
+ {
+ "name": "Manglal",
+ "pos_x": 13.59375,
+ "pos_y": 48.0625,
+ "pos_z": -37.03125,
+ "stations": "Lukyanenko Terminal,Grassmann Installation"
+ },
+ {
+ "name": "Mangpa",
+ "pos_x": -38.375,
+ "pos_y": 105.78125,
+ "pos_z": 64.15625,
+ "stations": "Vizcaino Vision,Cortes Refinery,Alvares Barracks"
+ },
+ {
+ "name": "Manguts",
+ "pos_x": 43.46875,
+ "pos_y": -5.96875,
+ "pos_z": -45.21875,
+ "stations": "Readdy Survey"
+ },
+ {
+ "name": "Mangwe",
+ "pos_x": 99.46875,
+ "pos_y": -3.25,
+ "pos_z": 25.71875,
+ "stations": "Onnes City,Bondar Dock,Laird Vista,Shaw Station,Sharipov Orbital,Spedding Dock,Smith Dock,Hoshide Enterprise,Morris' Inheritance"
+ },
+ {
+ "name": "Mangwutja",
+ "pos_x": 127.28125,
+ "pos_y": -132.3125,
+ "pos_z": -84,
+ "stations": "Pond Hub,Tousey Ring,Shapley Vision,Stuart Keep,Wilhelm Works"
+ },
+ {
+ "name": "Manhari",
+ "pos_x": 113.1875,
+ "pos_y": -3.15625,
+ "pos_z": -103,
+ "stations": "Sarmiento de Gamboa City,Strzelecki Colony,McMullen Port,Hill Vision,Lewitt Terminal"
+ },
+ {
+ "name": "Manhotti",
+ "pos_x": -118.90625,
+ "pos_y": -98.75,
+ "pos_z": -99.875,
+ "stations": "Gaiman Platform,Carlisle Settlement"
+ },
+ {
+ "name": "Manian",
+ "pos_x": 99.96875,
+ "pos_y": -89,
+ "pos_z": -11,
+ "stations": "Leopold Heckmann Settlement"
+ },
+ {
+ "name": "Manianscudo",
+ "pos_x": 62.09375,
+ "pos_y": -107.40625,
+ "pos_z": -24.28125,
+ "stations": "Miller Port,Ghez Orbital,al-Kashi Dock,Riess Terminal,Fife Enterprise,Palitzsch Hub,Andoyer Dock,Hartmann Gateway,Curtiss Port,Anderson Terminal,Pajdusakova Vista,Poyser Survey,Zwicky Beacon,Lewis Terminal"
+ },
+ {
+ "name": "Maniatetii",
+ "pos_x": 26.3125,
+ "pos_y": -101.65625,
+ "pos_z": 169.28125,
+ "stations": "Sei's Claim"
+ },
+ {
+ "name": "Manibozho",
+ "pos_x": 60.09375,
+ "pos_y": 33.625,
+ "pos_z": 100.375,
+ "stations": "Sullivan Reformatory,Aristotle Colony"
+ },
+ {
+ "name": "Manidone",
+ "pos_x": -141.75,
+ "pos_y": -65.15625,
+ "pos_z": 9.96875,
+ "stations": "Kroehl Port,Pike Terminal,Staden Colony"
+ },
+ {
+ "name": "Manie",
+ "pos_x": 107.40625,
+ "pos_y": -37.6875,
+ "pos_z": 83.125,
+ "stations": "Greenstein Estate,Dean Landing"
+ },
+ {
+ "name": "Manii",
+ "pos_x": -38.53125,
+ "pos_y": 83.1875,
+ "pos_z": 82.71875,
+ "stations": "Dana Settlement,Foda Vision,Scott Depot"
+ },
+ {
+ "name": "Manike",
+ "pos_x": -35.71875,
+ "pos_y": 7.90625,
+ "pos_z": 130.6875,
+ "stations": "Guin Orbital,Chern Penal colony,Filter Horizons"
+ },
+ {
+ "name": "Manikena",
+ "pos_x": -118.84375,
+ "pos_y": -104.21875,
+ "pos_z": 40.46875,
+ "stations": "Kazantsev Dock"
+ },
+ {
+ "name": "Manindini",
+ "pos_x": 34.1875,
+ "pos_y": -93.71875,
+ "pos_z": 158.03125,
+ "stations": "Ikeya Station,Pacheco Vision,Skjellerup Gateway,Finch Base,Ore Installation"
+ },
+ {
+ "name": "Manisi",
+ "pos_x": -148.15625,
+ "pos_y": 2.75,
+ "pos_z": 26.84375,
+ "stations": "Barba Station,Townshend Orbital,Robson Point"
+ },
+ {
+ "name": "Manissyet",
+ "pos_x": -92.96875,
+ "pos_y": -73.8125,
+ "pos_z": 21.53125,
+ "stations": "Stebler Ring,Jensen Station"
+ },
+ {
+ "name": "Manit",
+ "pos_x": -116.25,
+ "pos_y": 90.90625,
+ "pos_z": 29.1875,
+ "stations": "Hardy Terminal,Gaultier de Varennes Horizons,Finney City"
+ },
+ {
+ "name": "Manite",
+ "pos_x": -8.46875,
+ "pos_y": -19.21875,
+ "pos_z": 166.90625,
+ "stations": "Irens Dock,Stackpole Platform,Pinzon Orbital,Kneale Survey"
+ },
+ {
+ "name": "Manites",
+ "pos_x": 117.96875,
+ "pos_y": -94.40625,
+ "pos_z": -53.65625,
+ "stations": "Leonard Station,Backlund Hangar,Younghusband Survey"
+ },
+ {
+ "name": "Manitola",
+ "pos_x": 124.21875,
+ "pos_y": -106.78125,
+ "pos_z": 71.09375,
+ "stations": "Slipher Station,Beer Platform,Rigaux Beacon"
+ },
+ {
+ "name": "Manji",
+ "pos_x": -161.5,
+ "pos_y": 66.84375,
+ "pos_z": 25.15625,
+ "stations": "Nicholson Ring,Rosenberg Hub,Kelly Hub,Russell Beacon,Chilton Settlement"
+ },
+ {
+ "name": "Mankatha",
+ "pos_x": 170.1875,
+ "pos_y": -45.875,
+ "pos_z": 45.875,
+ "stations": "Truman Platform,Roemer Terminal,Tolstoi Beacon"
+ },
+ {
+ "name": "Mankob",
+ "pos_x": -84.78125,
+ "pos_y": 21.90625,
+ "pos_z": -44.40625,
+ "stations": "Shaw Orbital,Jones City,Dalmas Settlement"
+ },
+ {
+ "name": "Manktas",
+ "pos_x": -104.375,
+ "pos_y": 71.71875,
+ "pos_z": -56.03125,
+ "stations": "Fast Ring,Griffith Gateway,Rucker Gateway,Jiushao Depot,Lee Gateway,Capek Enterprise,MacVicar Ring,Chandler Port,Yolen City"
+ },
+ {
+ "name": "Mankul",
+ "pos_x": 12,
+ "pos_y": -120.1875,
+ "pos_z": -61,
+ "stations": "Kandrup Vision"
+ },
+ {
+ "name": "Mannboo",
+ "pos_x": 32.375,
+ "pos_y": 21.71875,
+ "pos_z": 93.34375,
+ "stations": "Hurston Terminal,Cenker Orbital,Halley Port,McDevitt Terminal,Pike Keep,Khrenov Survey"
+ },
+ {
+ "name": "Mannica",
+ "pos_x": -130.6875,
+ "pos_y": 2.78125,
+ "pos_z": 17.84375,
+ "stations": "Wankel City,Born Plant"
+ },
+ {
+ "name": "Mannodava",
+ "pos_x": -44.96875,
+ "pos_y": -51.46875,
+ "pos_z": 56.125,
+ "stations": "Ali Port,Spedding City,Moffitt Beacon,Maxwell Gateway,Hoard Point,Fleming Port,Carter Hub,Horowitz Hub,Clauss Gateway,Kidman Hub,Messerschmid City,Wisoff Terminal"
+ },
+ {
+ "name": "Mannona",
+ "pos_x": -79.875,
+ "pos_y": 40.09375,
+ "pos_z": 37.59375,
+ "stations": "Thornycroft Penal colony"
+ },
+ {
+ "name": "Mannones",
+ "pos_x": -45.28125,
+ "pos_y": -163.59375,
+ "pos_z": 73.03125,
+ "stations": "Tyson Landing"
+ },
+ {
+ "name": "Mannosuse",
+ "pos_x": -81.6875,
+ "pos_y": -122.46875,
+ "pos_z": -20.625,
+ "stations": "Bluford Beacon,Ron Hubbard's Inheritance"
+ },
+ {
+ "name": "Mannovalo",
+ "pos_x": -127.46875,
+ "pos_y": -79.65625,
+ "pos_z": 50,
+ "stations": "McHugh Orbital,Laird Survey,Andree Settlement"
+ },
+ {
+ "name": "Mannovichs",
+ "pos_x": 196.4375,
+ "pos_y": -145.625,
+ "pos_z": 80.03125,
+ "stations": "Mies van der Rohe Relay"
+ },
+ {
+ "name": "Mannovit",
+ "pos_x": -3.90625,
+ "pos_y": -21.75,
+ "pos_z": -122.25,
+ "stations": "Galouye Enterprise,Fischer Platform,Antonelli Terminal"
+ },
+ {
+ "name": "Mansmonir",
+ "pos_x": -26.4375,
+ "pos_y": 113.5,
+ "pos_z": -4.90625,
+ "stations": "Wilhelm Installation,Allen's Pride"
+ },
+ {
+ "name": "Mant",
+ "pos_x": 66.1875,
+ "pos_y": 132.53125,
+ "pos_z": -49.3125,
+ "stations": "Wylie Landing,Auer Settlement"
+ },
+ {
+ "name": "Mantani",
+ "pos_x": 142.59375,
+ "pos_y": 5.96875,
+ "pos_z": -2.1875,
+ "stations": "Foucault Dock"
+ },
+ {
+ "name": "Manten",
+ "pos_x": 45.53125,
+ "pos_y": 16.1875,
+ "pos_z": -119.75,
+ "stations": "Leonov Settlement,Bowen Orbital,Bering Depot"
+ },
+ {
+ "name": "Mantet",
+ "pos_x": -41.75,
+ "pos_y": 97.03125,
+ "pos_z": 78.34375,
+ "stations": "Lopez-Alegria Colony,Bykovsky Platform"
+ },
+ {
+ "name": "Manthe",
+ "pos_x": 63.78125,
+ "pos_y": -144.53125,
+ "pos_z": -22.875,
+ "stations": "Wirtanen Port,DiFate Silo"
+ },
+ {
+ "name": "Mantja",
+ "pos_x": -12.4375,
+ "pos_y": -32.875,
+ "pos_z": -71.0625,
+ "stations": "Currie Plant,McArthur Station"
+ },
+ {
+ "name": "Mantoac",
+ "pos_x": 41.75,
+ "pos_y": -167.8125,
+ "pos_z": 119.34375,
+ "stations": "Tully Orbital"
+ },
+ {
+ "name": "Mantobices",
+ "pos_x": 147.9375,
+ "pos_y": 76.78125,
+ "pos_z": -72.5625,
+ "stations": "Goulart Terminal,Robinson Platform,Gunn Enterprise,Perry Works,Reed Terminal"
+ },
+ {
+ "name": "Mantuate",
+ "pos_x": 58.40625,
+ "pos_y": -45.1875,
+ "pos_z": 67.75,
+ "stations": "Marriott Ring,Hadid Port,Oosterhoff Terminal,Scheerbart Prospect,Stein Enterprise"
+ },
+ {
+ "name": "Mantunt",
+ "pos_x": 141.5625,
+ "pos_y": -17.875,
+ "pos_z": 40.53125,
+ "stations": "Whitney Platform"
+ },
+ {
+ "name": "Mantxe",
+ "pos_x": -3.28125,
+ "pos_y": -8.375,
+ "pos_z": 58.75,
+ "stations": "de Sousa Ring,Parise Penal colony,Bennett Enterprise,Carpini Station"
+ },
+ {
+ "name": "Manukan",
+ "pos_x": -31.5625,
+ "pos_y": -74.5,
+ "pos_z": 156,
+ "stations": "Burton Terminal,Stein Orbital,Savorgnan de Brazza Enterprise,Clement City,Bao Works"
+ },
+ {
+ "name": "Manuki",
+ "pos_x": -77.375,
+ "pos_y": -68.3125,
+ "pos_z": 85.4375,
+ "stations": "Young's Claim,Garcia Landing"
+ },
+ {
+ "name": "Mao",
+ "pos_x": 86.21875,
+ "pos_y": 35.84375,
+ "pos_z": -133.25,
+ "stations": "Dickson Station,Alten Colony,Ford Depot"
+ },
+ {
+ "name": "Maohimr",
+ "pos_x": 9.1875,
+ "pos_y": 142.8125,
+ "pos_z": 84.21875,
+ "stations": "Burnet Horizons,Bradbury Base,Wul Horizons"
+ },
+ {
+ "name": "Maolabal",
+ "pos_x": -43.09375,
+ "pos_y": 4.125,
+ "pos_z": 162.625,
+ "stations": "Mitropoulos Colony,Clifford Survey"
+ },
+ {
+ "name": "Maolang",
+ "pos_x": 142.375,
+ "pos_y": 134.3125,
+ "pos_z": 45.59375,
+ "stations": "Temple Prospect,Sekowski Asylum"
+ },
+ {
+ "name": "Maon",
+ "pos_x": 35,
+ "pos_y": -179.8125,
+ "pos_z": 54.78125,
+ "stations": "Andoyer Mines,Gurshtein Station,Joy Enterprise"
+ },
+ {
+ "name": "Maona",
+ "pos_x": -4.375,
+ "pos_y": -207.21875,
+ "pos_z": 42.59375,
+ "stations": "Bowell Settlement"
+ },
+ {
+ "name": "Maopi",
+ "pos_x": 56,
+ "pos_y": -13.71875,
+ "pos_z": 78.84375,
+ "stations": "Chadwick Orbital,Lockhart Enterprise"
+ },
+ {
+ "name": "Maoriousta",
+ "pos_x": 61.8125,
+ "pos_y": -1.96875,
+ "pos_z": -143.8125,
+ "stations": "Mendeleev Base,Makarov Base,Varthema Terminal"
+ },
+ {
+ "name": "Maorsi",
+ "pos_x": 17.34375,
+ "pos_y": -126.6875,
+ "pos_z": 40.5,
+ "stations": "Karachkina City,Dowie Point,Oja Ring,Pittendreigh City"
+ },
+ {
+ "name": "Mapocori",
+ "pos_x": -115.5625,
+ "pos_y": 5.75,
+ "pos_z": -91.6875,
+ "stations": "Killough City,Mourelle Port,Ballard Station,Wood Depot,Libby Terminal,Hilbert Terminal"
+ },
+ {
+ "name": "Mapod",
+ "pos_x": 96.21875,
+ "pos_y": -148.15625,
+ "pos_z": 107.15625,
+ "stations": "Mil City,Panshin Silo,Rolland Prospect"
+ },
+ {
+ "name": "Mapon",
+ "pos_x": 145.6875,
+ "pos_y": 18.40625,
+ "pos_z": -90.78125,
+ "stations": "Searfoss Terminal,Mills Enterprise,Harding Depot"
+ },
+ {
+ "name": "Mapor",
+ "pos_x": -54.03125,
+ "pos_y": -75.46875,
+ "pos_z": 142.59375,
+ "stations": "Clayton Horizons,Nicholson Penal colony,Chaviano Gateway,Windt Enterprise,Ford Terminal"
+ },
+ {
+ "name": "Mapotepa",
+ "pos_x": -14.09375,
+ "pos_y": -112.84375,
+ "pos_z": 30.8125,
+ "stations": "Galle Landing,Jones Relay"
+ },
+ {
+ "name": "Mara",
+ "pos_x": 42.0625,
+ "pos_y": -96.375,
+ "pos_z": 57.90625,
+ "stations": "Richer City,Merritt Port,Lange Vision"
+ },
+ {
+ "name": "Maragi",
+ "pos_x": -18.15625,
+ "pos_y": -200.625,
+ "pos_z": 1.0625,
+ "stations": "Fox Survey"
+ },
+ {
+ "name": "Marahli",
+ "pos_x": 51.28125,
+ "pos_y": 59.8125,
+ "pos_z": -42.0625,
+ "stations": "Poincare Platform,Hand Lab"
+ },
+ {
+ "name": "Marahma",
+ "pos_x": 138.78125,
+ "pos_y": 47.34375,
+ "pos_z": 60.875,
+ "stations": "Timofeyevich Installation,Milnor Penal colony"
+ },
+ {
+ "name": "Marajoara",
+ "pos_x": 0,
+ "pos_y": -40.5625,
+ "pos_z": -73.90625,
+ "stations": "Vlamingh's Progress,Andrews Refinery"
+ },
+ {
+ "name": "Marakhi",
+ "pos_x": 114.78125,
+ "pos_y": 87.375,
+ "pos_z": -61.40625,
+ "stations": "Dickson Port,Johnson Orbital"
+ },
+ {
+ "name": "Maramovoy",
+ "pos_x": 47.21875,
+ "pos_y": -21.53125,
+ "pos_z": 126.3125,
+ "stations": "Melnick Gateway,Britnev Prospect,Due Observatory"
+ },
+ {
+ "name": "Marana",
+ "pos_x": 39.53125,
+ "pos_y": -57.875,
+ "pos_z": -70.40625,
+ "stations": "Forrester Port,Ramanujan Terminal,Christopher Legacy"
+ },
+ {
+ "name": "Maranganji",
+ "pos_x": 92.9375,
+ "pos_y": 4.875,
+ "pos_z": 148.34375,
+ "stations": "Burkin Platform,Barnaby Port,Maire Horizons"
+ },
+ {
+ "name": "Maranunggu",
+ "pos_x": 135.5,
+ "pos_y": -41.21875,
+ "pos_z": 37.3125,
+ "stations": "Forest Stop"
+ },
+ {
+ "name": "Mararri",
+ "pos_x": 130.84375,
+ "pos_y": -109.78125,
+ "pos_z": 76.1875,
+ "stations": "Eggen Point"
+ },
+ {
+ "name": "Marasing",
+ "pos_x": -89.03125,
+ "pos_y": 31.09375,
+ "pos_z": -11.15625,
+ "stations": "Landsteiner Terminal,Hawley Point,Khan Observatory,Kizim Dock"
+ },
+ {
+ "name": "Maratarini",
+ "pos_x": -106.5,
+ "pos_y": -45.15625,
+ "pos_z": 136.8125,
+ "stations": "Frechet Dock,Malcolm's Folly,Broderick Vision"
+ },
+ {
+ "name": "Maratians",
+ "pos_x": -88.8125,
+ "pos_y": -44.84375,
+ "pos_z": -70.25,
+ "stations": "Garriott Refinery,Anders Landing"
+ },
+ {
+ "name": "Maraudi",
+ "pos_x": 79.1875,
+ "pos_y": 58.28125,
+ "pos_z": 58,
+ "stations": "Hahn Hub,Stott Dock,Ellis Ring,Harding Bastion,Camarda Terminal"
+ },
+ {
+ "name": "Maray",
+ "pos_x": 142.09375,
+ "pos_y": -73.3125,
+ "pos_z": 71.28125,
+ "stations": "Leonard Sanctuary"
+ },
+ {
+ "name": "Mard",
+ "pos_x": -58.34375,
+ "pos_y": 16.25,
+ "pos_z": 137.53125,
+ "stations": "Womack Prospect"
+ },
+ {
+ "name": "Mardgrivik",
+ "pos_x": 81.25,
+ "pos_y": -7.9375,
+ "pos_z": 141.6875,
+ "stations": "Spring Dock,Horch Beacon"
+ },
+ {
+ "name": "Marditj",
+ "pos_x": 32.4375,
+ "pos_y": 15.0625,
+ "pos_z": 9.375,
+ "stations": "Liwei Platform,McCormick Terminal,Alexander Beacon"
+ },
+ {
+ "name": "Marduk",
+ "pos_x": -20.8125,
+ "pos_y": -12.75,
+ "pos_z": -43.875,
+ "stations": "Port Sippar,Amar Station"
+ },
+ {
+ "name": "Mari",
+ "pos_x": 22.5,
+ "pos_y": -12.4375,
+ "pos_z": 27.53125,
+ "stations": "Guidoni Hub"
+ },
+ {
+ "name": "Maria",
+ "pos_x": 24.625,
+ "pos_y": -98.46875,
+ "pos_z": -91.375,
+ "stations": "Zindell's Inheritance,Flynn Outpost"
+ },
+ {
+ "name": "Maria Bhuti",
+ "pos_x": 60.03125,
+ "pos_y": -240.96875,
+ "pos_z": 41.15625,
+ "stations": "van de Sande Bakhuyzen Station,Ohain Dock,Lagerkvist Vision,Brunner Town"
+ },
+ {
+ "name": "Marian Nungari",
+ "pos_x": -0.40625,
+ "pos_y": -140.625,
+ "pos_z": 43.75,
+ "stations": "West Orbital,Hirayama Base,Shaw Point"
+ },
+ {
+ "name": "Mariang",
+ "pos_x": 109.25,
+ "pos_y": -35.8125,
+ "pos_z": 132.65625,
+ "stations": "Wilson Landing,Mrkos Colony,Rocklynne Works"
+ },
+ {
+ "name": "Mariarna",
+ "pos_x": 33.625,
+ "pos_y": 33.8125,
+ "pos_z": 126,
+ "stations": "Corte-Real Hub,Frechet Gateway,van Vogt Dock,Fraas Horizons,Sterling Depot"
+ },
+ {
+ "name": "Maribe",
+ "pos_x": -140.75,
+ "pos_y": -85.21875,
+ "pos_z": -13.9375,
+ "stations": "Sarmiento de Gamboa Dock"
+ },
+ {
+ "name": "Maribone",
+ "pos_x": 35.0625,
+ "pos_y": 77.0625,
+ "pos_z": 98.9375,
+ "stations": "Nesvadba Colony"
+ },
+ {
+ "name": "Maric",
+ "pos_x": 30.65625,
+ "pos_y": -183.53125,
+ "pos_z": 56.1875,
+ "stations": "Janjetov Reach"
+ },
+ {
+ "name": "Marichi",
+ "pos_x": 128.90625,
+ "pos_y": -22.1875,
+ "pos_z": 81.09375,
+ "stations": "Whitworth Port,Foale Enterprise"
+ },
+ {
+ "name": "Marici",
+ "pos_x": 28.125,
+ "pos_y": -41.21875,
+ "pos_z": -15.59375,
+ "stations": "Gidzenko Dock,Khan Installation,Bakewell Enterprise"
+ },
+ {
+ "name": "Marico",
+ "pos_x": 85.3125,
+ "pos_y": -212.4375,
+ "pos_z": -57.8125,
+ "stations": "Arzachel Station,William Sargent Station,Navigator Observatory"
+ },
+ {
+ "name": "Maricoco",
+ "pos_x": 84.90625,
+ "pos_y": -40.8125,
+ "pos_z": 152.125,
+ "stations": "Lopez's Claim,Kuchner's Folly"
+ },
+ {
+ "name": "Maricoriang",
+ "pos_x": -1.40625,
+ "pos_y": 19.96875,
+ "pos_z": 136.53125,
+ "stations": "Sekowski Dock,Slusser Port,Jenkinson Port,Mallory Prospect"
+ },
+ {
+ "name": "Maridal",
+ "pos_x": -53.78125,
+ "pos_y": -22.375,
+ "pos_z": 39.09375,
+ "stations": "Vonarburg Port,Dedman Gateway"
+ },
+ {
+ "name": "Maridjabin",
+ "pos_x": 57.78125,
+ "pos_y": -2.65625,
+ "pos_z": -64.3125,
+ "stations": "Kerr Hub,Jacquard Beacon"
+ },
+ {
+ "name": "Maridwyn",
+ "pos_x": 90.46875,
+ "pos_y": 16.40625,
+ "pos_z": 21.625,
+ "stations": "Pashin Port,Teng Prospect,Anderson Station,Werber Station,Hand Orbital,Soto Station,Vizcaino Orbital,Friend Colony,Rothman Dock,Cousteau Vision,Killough Dock,Green City"
+ },
+ {
+ "name": "Mariga",
+ "pos_x": 42.34375,
+ "pos_y": -174.71875,
+ "pos_z": 101.84375,
+ "stations": "Lavochkin Terminal,Witt Mines,Hulse Station,Pollas Keep,Gold Prospect"
+ },
+ {
+ "name": "Marigo",
+ "pos_x": 85.4375,
+ "pos_y": 96.96875,
+ "pos_z": -28.28125,
+ "stations": "Junlong Gateway"
+ },
+ {
+ "name": "Marijemala",
+ "pos_x": -138.96875,
+ "pos_y": 67.84375,
+ "pos_z": 2.65625,
+ "stations": "Ayerdhal Beacon"
+ },
+ {
+ "name": "Mariko",
+ "pos_x": 126,
+ "pos_y": 95.59375,
+ "pos_z": 106.5,
+ "stations": "Tshang Retreat,Langsdorff Vision"
+ },
+ {
+ "name": "Marimpt",
+ "pos_x": -49.90625,
+ "pos_y": -119.625,
+ "pos_z": 50.90625,
+ "stations": "Cartmill Terminal,Williams Port,Longyear Mines,Humphreys Port,Verrazzano's Progress"
+ },
+ {
+ "name": "Marindhs",
+ "pos_x": -161.6875,
+ "pos_y": -56.375,
+ "pos_z": -22.875,
+ "stations": "Meitner Holdings,Virts Dock"
+ },
+ {
+ "name": "Marines",
+ "pos_x": 106.3125,
+ "pos_y": -104.78125,
+ "pos_z": 90.25,
+ "stations": "Friedman Ring,Carpenter Dock,Saarinen Terminal,Villarceau Enterprise,McMillan Orbital"
+ },
+ {
+ "name": "Maring",
+ "pos_x": 64.59375,
+ "pos_y": -9.1875,
+ "pos_z": -94.0625,
+ "stations": "Reis Gateway,Phillifent Terminal,Rawn Port"
+ },
+ {
+ "name": "Maringpho",
+ "pos_x": -27.59375,
+ "pos_y": 156.75,
+ "pos_z": -9.25,
+ "stations": "Forfait Legacy"
+ },
+ {
+ "name": "Maris",
+ "pos_x": 102.25,
+ "pos_y": -90.15625,
+ "pos_z": 19.84375,
+ "stations": "Hooker Dock,Reeves Installation,Carpini Hub"
+ },
+ {
+ "name": "Maritai",
+ "pos_x": -39.28125,
+ "pos_y": -182.375,
+ "pos_z": 40.75,
+ "stations": "Vaisala Mines"
+ },
+ {
+ "name": "Mariterni",
+ "pos_x": 150.40625,
+ "pos_y": -145.65625,
+ "pos_z": 132.0625,
+ "stations": "Goodricke Landing,Whipple Landing,Emshwiller Vision,Hambly Landing"
+ },
+ {
+ "name": "Maritou",
+ "pos_x": -3.34375,
+ "pos_y": -175.25,
+ "pos_z": -31.78125,
+ "stations": "Fan Survey,Harvia Mines"
+ },
+ {
+ "name": "Maritzambwe",
+ "pos_x": 67.15625,
+ "pos_y": -154.21875,
+ "pos_z": -82.78125,
+ "stations": "Whipple Camp"
+ },
+ {
+ "name": "Mariyacoch",
+ "pos_x": -64.28125,
+ "pos_y": -41.78125,
+ "pos_z": 27.9375,
+ "stations": "Kaku Port,Savitskaya Station,Chamitoff Dock,Whitney Hub,Pontes Terminal,Priestley Station,Bujold Prospect,Bloomfield Port,Hoffman Depot,Byrd Legacy"
+ },
+ {
+ "name": "Mariyota",
+ "pos_x": 122.59375,
+ "pos_y": -128.03125,
+ "pos_z": 156.3125,
+ "stations": "Lee Terminal,Tsiolkovsky Oasis,Piazzi Beacon"
+ },
+ {
+ "name": "Marizi",
+ "pos_x": -34.9375,
+ "pos_y": 39.90625,
+ "pos_z": -79.5,
+ "stations": "Thiele Dock,Culpeper Terminal,Culpeper Station,Wilcutt Dock,Landsteiner Dock,Elcano Barracks,Lewis Holdings,Lazarev Orbital,Drexler Port,Chilton Gateway,Goeppert-Mayer Port,Lewis Base,Bischoff Installation,Pinzon Terminal"
+ },
+ {
+ "name": "Markab",
+ "pos_x": -101.46875,
+ "pos_y": -86.5625,
+ "pos_z": 1.5,
+ "stations": "Perrin Port,Remec Survey"
+ },
+ {
+ "name": "Markathun",
+ "pos_x": -120.875,
+ "pos_y": 35.28125,
+ "pos_z": 39.40625,
+ "stations": "Bramah Stop,McCulley Beacon"
+ },
+ {
+ "name": "Marki",
+ "pos_x": 81.5,
+ "pos_y": -84.9375,
+ "pos_z": 44.28125,
+ "stations": "Ahnert-Rohlfs Terminal,Cartier Survey,Crossfield Market,Yanai Enterprise"
+ },
+ {
+ "name": "Markin",
+ "pos_x": -36.375,
+ "pos_y": -69.6875,
+ "pos_z": 109.5625,
+ "stations": "Fossey Terminal,Sanger Legacy,Lawhead Base"
+ },
+ {
+ "name": "Marku",
+ "pos_x": 18.125,
+ "pos_y": 115.4375,
+ "pos_z": -123.8125,
+ "stations": "Reed Point,Sagan Landing,Boucher Prospect"
+ },
+ {
+ "name": "Marmatae",
+ "pos_x": -99.21875,
+ "pos_y": -92.84375,
+ "pos_z": -84.03125,
+ "stations": "Alexander Refinery,Cremona Platform,Filter's Progress"
+ },
+ {
+ "name": "Maro",
+ "pos_x": 51,
+ "pos_y": 24.75,
+ "pos_z": 73.625,
+ "stations": "Karl Diesel Colony,Wrangell Outpost"
+ },
+ {
+ "name": "Marogee",
+ "pos_x": 53.78125,
+ "pos_y": -126.96875,
+ "pos_z": 72.21875,
+ "stations": "Siegel Hub,Watson Orbital,Neumann Reformatory"
+ },
+ {
+ "name": "Maroi",
+ "pos_x": -16.0625,
+ "pos_y": 2.75,
+ "pos_z": -170.5,
+ "stations": "Lucid Station,Beregovoi Orbital,Bulleid City,Stjepan Seljan Refinery,Hornby Works"
+ },
+ {
+ "name": "Maroine",
+ "pos_x": 107.375,
+ "pos_y": 6.4375,
+ "pos_z": 45.25,
+ "stations": "Garden Vision,Bates Vision,Katzenstein Survey"
+ },
+ {
+ "name": "Marojini",
+ "pos_x": -99.84375,
+ "pos_y": 34.46875,
+ "pos_z": -9.3125,
+ "stations": "Hackworth Terminal,Treshchov Ring,Burton's Progress,Sinclair Port,Carpini Works,Preuss Survey"
+ },
+ {
+ "name": "Marowalan",
+ "pos_x": 8.0625,
+ "pos_y": -94.78125,
+ "pos_z": 10.875,
+ "stations": "Kuipers Orbital,Carter City,Andreas Point"
+ },
+ {
+ "name": "Marra",
+ "pos_x": 173.6875,
+ "pos_y": 57.25,
+ "pos_z": -16.4375,
+ "stations": "MacLeod Colony,Davis Enterprise,Fraser Installation,Anthony Prospect"
+ },
+ {
+ "name": "Marrago",
+ "pos_x": -51.84375,
+ "pos_y": -133.75,
+ "pos_z": 95.8125,
+ "stations": "Sheckley Terminal,Trimble Orbital"
+ },
+ {
+ "name": "Marrallang",
+ "pos_x": 86.0625,
+ "pos_y": -149.3125,
+ "pos_z": 48.28125,
+ "stations": "Pajdusakova Gateway,Busemann Gateway,Stromgren Dock,Coggia Terminal,Alfven Hub,Wiener Horizons,McMillan Ring,Orbik Gateway,Naubakht Observatory,Kawasato Silo,Beltrami's Inheritance,Hatanaka Dock,Anderson Station"
+ },
+ {
+ "name": "Marralteki",
+ "pos_x": -56.03125,
+ "pos_y": 80.1875,
+ "pos_z": 72.46875,
+ "stations": "al-Din Port,Bushnell Terminal,Joule Hub,Jett Dock,Kovalyonok Enterprise,Bamford Enterprise,Watson Orbital,Hopkins Arsenal,Priestley Vista,Jones Exchange"
+ },
+ {
+ "name": "Marrenses",
+ "pos_x": 104.71875,
+ "pos_y": -13.46875,
+ "pos_z": 85.59375,
+ "stations": "Biggle Station"
+ },
+ {
+ "name": "Marrivits",
+ "pos_x": 69.40625,
+ "pos_y": -31.5,
+ "pos_z": 143.65625,
+ "stations": "Matheson Dock,Lewitt Point,Sheckley Ring"
+ },
+ {
+ "name": "Marsinatani",
+ "pos_x": -87.21875,
+ "pos_y": 40.34375,
+ "pos_z": -9.5,
+ "stations": "Gernsback Survey,Capek Prospect,Swift Prospect"
+ },
+ {
+ "name": "Marson",
+ "pos_x": -29.21875,
+ "pos_y": -38.53125,
+ "pos_z": -78.96875,
+ "stations": "Rushworth Horizons"
+ },
+ {
+ "name": "Marsonna",
+ "pos_x": -76.21875,
+ "pos_y": -118.46875,
+ "pos_z": -82.625,
+ "stations": "Knight Landing,Kneale Landing"
+ },
+ {
+ "name": "Marsuk",
+ "pos_x": 32.59375,
+ "pos_y": 101.875,
+ "pos_z": 21.15625,
+ "stations": "Neff Sanctuary,Maclaurin Point"
+ },
+ {
+ "name": "Martio",
+ "pos_x": 151.84375,
+ "pos_y": -85.09375,
+ "pos_z": 26.25,
+ "stations": "Kuchemann Settlement,Dufay Station,Piazzi Port,Schmidt Plant,Bartolomeu de Gusmao's Folly"
+ },
+ {
+ "name": "Martudja",
+ "pos_x": -134.75,
+ "pos_y": -28.71875,
+ "pos_z": 103.84375,
+ "stations": "Bugrov Point"
+ },
+ {
+ "name": "Marubal",
+ "pos_x": 59.5625,
+ "pos_y": -0.78125,
+ "pos_z": 75.78125,
+ "stations": "Gernsback Works,Priestley Dock,Bowen City,Tani Gateway"
+ },
+ {
+ "name": "Maruda",
+ "pos_x": -12.125,
+ "pos_y": -13.15625,
+ "pos_z": -184.5625,
+ "stations": "Murphy Landing,Lerman Vision"
+ },
+ {
+ "name": "Marule",
+ "pos_x": 128.875,
+ "pos_y": -133.0625,
+ "pos_z": 32.96875,
+ "stations": "Rosenberger Dock,Sopwith Vista"
+ },
+ {
+ "name": "Marulta",
+ "pos_x": -52.8125,
+ "pos_y": -24,
+ "pos_z": -131.09375,
+ "stations": "Baturin Hub,Sinclair City,Alexandria Enterprise,Junlong Orbital,Reilly Depot"
+ },
+ {
+ "name": "Maruti",
+ "pos_x": -121.84375,
+ "pos_y": 119.40625,
+ "pos_z": 15.4375,
+ "stations": "Jacobi Stop,Liouville Point,Monge Settlement"
+ },
+ {
+ "name": "Maruts",
+ "pos_x": 169.15625,
+ "pos_y": 31.90625,
+ "pos_z": 38.71875,
+ "stations": "Gelfand Enterprise,Dupuy de Lome Installation,Brooks Installation"
+ },
+ {
+ "name": "Marya Wang",
+ "pos_x": 107.875,
+ "pos_y": -79.125,
+ "pos_z": -0.1875,
+ "stations": "Savorgnan de Brazza Port,Cartan Port,Marusek Depot"
+ },
+ {
+ "name": "Masaai",
+ "pos_x": -47.875,
+ "pos_y": -0.875,
+ "pos_z": 19.5625,
+ "stations": "Euler Hub,Dana Landing"
+ },
+ {
+ "name": "Masai",
+ "pos_x": -138.90625,
+ "pos_y": 52.75,
+ "pos_z": -85.75,
+ "stations": "Sinclair Port"
+ },
+ {
+ "name": "Masans",
+ "pos_x": 64.90625,
+ "pos_y": 19.71875,
+ "pos_z": -40.53125,
+ "stations": "Wang City"
+ },
+ {
+ "name": "Masar",
+ "pos_x": 133.6875,
+ "pos_y": -248.53125,
+ "pos_z": 86.53125,
+ "stations": "Polya Lab,Bingzhen Survey"
+ },
+ {
+ "name": "Mashian",
+ "pos_x": 64.40625,
+ "pos_y": -4.21875,
+ "pos_z": -153.65625,
+ "stations": "Peirce Holdings,Bretnor Bastion,Lerner Station"
+ },
+ {
+ "name": "Massagetae",
+ "pos_x": -83.65625,
+ "pos_y": -23.71875,
+ "pos_z": -139.8125,
+ "stations": "McMullen Vision"
+ },
+ {
+ "name": "Masses",
+ "pos_x": -35.09375,
+ "pos_y": 1.96875,
+ "pos_z": -126.8125,
+ "stations": "Khan Enterprise,Bosch Terminal,Bear Laboratory"
+ },
+ {
+ "name": "Masszony",
+ "pos_x": 36.34375,
+ "pos_y": 5.75,
+ "pos_z": 38.3125,
+ "stations": "Pashin Settlement"
+ },
+ {
+ "name": "Mat Zemlya",
+ "pos_x": 37.03125,
+ "pos_y": -56.5,
+ "pos_z": 24.71875,
+ "stations": "Pelliot Vision,Tanner Dock"
+ },
+ {
+ "name": "Mata",
+ "pos_x": 38.625,
+ "pos_y": -0.5,
+ "pos_z": 16.53125,
+ "stations": "Cayley Gateway"
+ },
+ {
+ "name": "Mata Athi",
+ "pos_x": 69.09375,
+ "pos_y": 114.6875,
+ "pos_z": -106.53125,
+ "stations": "Duffy Horizons,Zamka Base"
+ },
+ {
+ "name": "Matec",
+ "pos_x": 62.84375,
+ "pos_y": -118.78125,
+ "pos_z": -10.5625,
+ "stations": "Wafa Enterprise,Guth Enterprise"
+ },
+ {
+ "name": "Mati",
+ "pos_x": 156.90625,
+ "pos_y": -137.0625,
+ "pos_z": 26.4375,
+ "stations": "Bickel Dock,Schiaparelli Station,Scotti Port,Arnold's Pride,Bailly Installation"
+ },
+ {
+ "name": "Mati Chuqui",
+ "pos_x": 14.71875,
+ "pos_y": -194.6875,
+ "pos_z": 77.21875,
+ "stations": "Ban Holdings,Kobayashi Terminal,Shavyrin Orbital,Lopez Port"
+ },
+ {
+ "name": "Matia",
+ "pos_x": 115.03125,
+ "pos_y": -59.0625,
+ "pos_z": 20.40625,
+ "stations": "Gustav Sporer Market,Laird's Progress"
+ },
+ {
+ "name": "Matiku",
+ "pos_x": 118.96875,
+ "pos_y": -176.40625,
+ "pos_z": 34.65625,
+ "stations": "Asclepi Port,Bertin Barracks,Safdie Hub"
+ },
+ {
+ "name": "Matikuoluk",
+ "pos_x": 25.15625,
+ "pos_y": -44.9375,
+ "pos_z": 73.15625,
+ "stations": "Feoktistov Vista,Oluwafemi Terminal,Binet Hub,Rutherford City,Szebehely Dock,al-Khowarizmi Keep,Hopkinson Enterprise,Armstrong Orbital,Pierres' Claim,Roberts Base,Morgan Survey"
+ },
+ {
+ "name": "Matio",
+ "pos_x": 85.0625,
+ "pos_y": -24,
+ "pos_z": -15.25,
+ "stations": "Tayler Relay,Hale Horizons,Higginbotham Enterprise"
+ },
+ {
+ "name": "Matipu",
+ "pos_x": -12.0625,
+ "pos_y": 154.28125,
+ "pos_z": 26.25,
+ "stations": "Judson City,Bordage Vision,Gessi Landing,Krylov Hub"
+ },
+ {
+ "name": "Matire",
+ "pos_x": 141.40625,
+ "pos_y": -151.21875,
+ "pos_z": -18.03125,
+ "stations": "Henderson's Inheritance"
+ },
+ {
+ "name": "Matlehi",
+ "pos_x": -87.96875,
+ "pos_y": -18.6875,
+ "pos_z": 85.8125,
+ "stations": "Markov Enterprise,Daniel Terminal,Bunnell Ring,Sellings Gateway,Hinz Camp,Kube-McDowell Port,Piccard Port,Lee Depot,Verrazzano Vision"
+ },
+ {
+ "name": "Matres",
+ "pos_x": 29.375,
+ "pos_y": 59.46875,
+ "pos_z": 24.5,
+ "stations": "Malerba Survey,Fujimori Point"
+ },
+ {
+ "name": "Matsa",
+ "pos_x": -106.125,
+ "pos_y": -41.46875,
+ "pos_z": 61.3125,
+ "stations": "Kirk Refinery"
+ },
+ {
+ "name": "Matses",
+ "pos_x": -98.1875,
+ "pos_y": -24.53125,
+ "pos_z": 88.25,
+ "stations": "Manarov Works"
+ },
+ {
+ "name": "Matshiru",
+ "pos_x": 94.75,
+ "pos_y": -69.34375,
+ "pos_z": -104.0625,
+ "stations": "Lopez-Alegria Works"
+ },
+ {
+ "name": "Matsucha",
+ "pos_x": -49.46875,
+ "pos_y": -107.1875,
+ "pos_z": -100.6875,
+ "stations": "Moore Works,Bartlett Depot,Menzies Platform"
+ },
+ {
+ "name": "Matsya",
+ "pos_x": -79.09375,
+ "pos_y": -120.46875,
+ "pos_z": -83.34375,
+ "stations": "Pawelczyk Orbital,Singer Orbital"
+ },
+ {
+ "name": "Matucae",
+ "pos_x": 98.90625,
+ "pos_y": -170.625,
+ "pos_z": 19.15625,
+ "stations": "Somerville Orbital,Dumont Survey,Sheremetevsky Terminal,Sitterly Base"
+ },
+ {
+ "name": "Matucanth",
+ "pos_x": -72.1875,
+ "pos_y": -105.875,
+ "pos_z": 27.78125,
+ "stations": "Sagan Orbital,Robinson Station,Battuta Port,Illy Enterprise,Ericsson Ring,McKay Enterprise,Irvin Dock"
+ },
+ {
+ "name": "Matunus",
+ "pos_x": -75.375,
+ "pos_y": 31.71875,
+ "pos_z": -100.75,
+ "stations": "Hieb Settlement,Sharipov Terminal,Lavoisier Enterprise"
+ },
+ {
+ "name": "Maturics",
+ "pos_x": -4.25,
+ "pos_y": -34.875,
+ "pos_z": 99.125,
+ "stations": "Lawson Ring"
+ },
+ {
+ "name": "Maturina",
+ "pos_x": 179.84375,
+ "pos_y": -133.625,
+ "pos_z": 2.1875,
+ "stations": "Lockwood's Claim,Green's Folly"
+ },
+ {
+ "name": "Matuveani",
+ "pos_x": -35.03125,
+ "pos_y": -150.375,
+ "pos_z": 36.90625,
+ "stations": "Puiseux Landing,Gilliland Barracks,Dean Orbital,Isaev Platform"
+ },
+ {
+ "name": "Matuveltan",
+ "pos_x": -103.6875,
+ "pos_y": -4.59375,
+ "pos_z": -120.625,
+ "stations": "Irens Beacon"
+ },
+ {
+ "name": "Matyar",
+ "pos_x": 69.25,
+ "pos_y": 113.0625,
+ "pos_z": 58.1875,
+ "stations": "Stillman Station,Witt Station,Shatner Terminal,Arnarson Enterprise,Naddoddur Terminal,Klink Landing,Robinson's Progress,Fernandez Installation,Bass Relay"
+ },
+ {
+ "name": "Mau",
+ "pos_x": -19.875,
+ "pos_y": 24.875,
+ "pos_z": -119.9375,
+ "stations": "Sheffield Hub,Temple Hub,Rice City,Bulychev Station,Kummer Port,Maybury Prospect,Kennan Colony,Scott Beacon"
+ },
+ {
+ "name": "Maujas",
+ "pos_x": -53.59375,
+ "pos_y": -115.875,
+ "pos_z": -26.0625,
+ "stations": "Glazkov Hangar"
+ },
+ {
+ "name": "Maujavant",
+ "pos_x": -48.0625,
+ "pos_y": 16.71875,
+ "pos_z": -44.9375,
+ "stations": "Jordan Settlement,Rose Base,Campbell Settlement"
+ },
+ {
+ "name": "Maujinagoto",
+ "pos_x": -77.46875,
+ "pos_y": 16.09375,
+ "pos_z": -19.5625,
+ "stations": "Lubin Installation,Gooch Hub,Reilly Settlement"
+ },
+ {
+ "name": "Maungbira",
+ "pos_x": 158.78125,
+ "pos_y": -22.9375,
+ "pos_z": 27.65625,
+ "stations": "Nomen's Claim"
+ },
+ {
+ "name": "Maunggu",
+ "pos_x": 73.875,
+ "pos_y": -101.59375,
+ "pos_z": 0.40625,
+ "stations": "van Vogt City,Morgan Terminal,Kennan Prospect,Patterson Silo,Waldrop Beacon"
+ },
+ {
+ "name": "Mauninovit",
+ "pos_x": 30.0625,
+ "pos_y": 31.78125,
+ "pos_z": 168.5625,
+ "stations": "Sevastyanov Orbital,Lazutkin Depot"
+ },
+ {
+ "name": "Maurr",
+ "pos_x": 50.59375,
+ "pos_y": -44.40625,
+ "pos_z": -66.75,
+ "stations": "Roberts Beacon,Kepler Platform,Bondar Platform"
+ },
+ {
+ "name": "Mawa",
+ "pos_x": -20.34375,
+ "pos_y": 76.5,
+ "pos_z": -134.25,
+ "stations": "Salgari Enterprise,Andersson Installation,Clapperton Holdings,Lopez-Alegria Depot"
+ },
+ {
+ "name": "Mawai",
+ "pos_x": -11.875,
+ "pos_y": 6.21875,
+ "pos_z": 157.0625,
+ "stations": "Barba Port"
+ },
+ {
+ "name": "Mawalivun",
+ "pos_x": -50.125,
+ "pos_y": -117,
+ "pos_z": 55.09375,
+ "stations": "Kinsey Depot"
+ },
+ {
+ "name": "Mawambi",
+ "pos_x": 65.1875,
+ "pos_y": 41.875,
+ "pos_z": 3.90625,
+ "stations": "Ostwald Hub"
+ },
+ {
+ "name": "Mawannona",
+ "pos_x": 172.5,
+ "pos_y": -93.25,
+ "pos_z": 69,
+ "stations": "Baillaud Dock,Kemurdzhian Prospect,Merchiston Base,Jones Observatory"
+ },
+ {
+ "name": "Mawasi",
+ "pos_x": 17.25,
+ "pos_y": -64.875,
+ "pos_z": -97.40625,
+ "stations": "Mayr Relay,Yang Terminal"
+ },
+ {
+ "name": "Mawu",
+ "pos_x": -4.78125,
+ "pos_y": 43.1875,
+ "pos_z": -27.3125,
+ "stations": "Dirac Barracks,Underwood Port,Ross Station"
+ },
+ {
+ "name": "Mawul",
+ "pos_x": 0.5625,
+ "pos_y": -137.84375,
+ "pos_z": 54.96875,
+ "stations": "Urkovic City,Yu Relay"
+ },
+ {
+ "name": "Mawula",
+ "pos_x": 114.28125,
+ "pos_y": -248,
+ "pos_z": 41.5,
+ "stations": "Melotte Mines,al-Kashi Survey,Danvers Depot"
+ },
+ {
+ "name": "Mawuru",
+ "pos_x": -172.21875,
+ "pos_y": 40.71875,
+ "pos_z": 80.9375,
+ "stations": "McAuley Platform"
+ },
+ {
+ "name": "Mawurunche",
+ "pos_x": 120,
+ "pos_y": -3.53125,
+ "pos_z": -13.90625,
+ "stations": "Mastracchio Settlement,Piper Mines,Lehtonen Holdings"
+ },
+ {
+ "name": "Maya",
+ "pos_x": -5.375,
+ "pos_y": 42.65625,
+ "pos_z": -58.46875,
+ "stations": "Duffy Dock,Hawking Ring,Barnes Enterprise,Chern Vision,Perrin Enterprise,Buckell Arsenal,Nixon's Inheritance,Fisher Enterprise,McArthur Terminal,Sohl Lab,Strekalov Station"
+ },
+ {
+ "name": "Mayaco",
+ "pos_x": -61.5,
+ "pos_y": 115.375,
+ "pos_z": 21.28125,
+ "stations": "Rocklynne Orbital,Bear Platform,Serre's Folly"
+ },
+ {
+ "name": "Mayala",
+ "pos_x": -122.75,
+ "pos_y": -19.0625,
+ "pos_z": -23.65625,
+ "stations": "Borlaug Enterprise"
+ },
+ {
+ "name": "Mayang",
+ "pos_x": 27.1875,
+ "pos_y": -207.875,
+ "pos_z": 27.21875,
+ "stations": "Mikulin Market,Cerulli Gateway,Hariot Port"
+ },
+ {
+ "name": "Mayapok",
+ "pos_x": 48.53125,
+ "pos_y": -182.90625,
+ "pos_z": -19.96875,
+ "stations": "Biesbroeck Station"
+ },
+ {
+ "name": "Mayaram",
+ "pos_x": 149.09375,
+ "pos_y": -110.0625,
+ "pos_z": 58.75,
+ "stations": "Kirkwood Dock,O'Leary Lab"
+ },
+ {
+ "name": "Mayoquot",
+ "pos_x": 96.9375,
+ "pos_y": -143.375,
+ "pos_z": 6.59375,
+ "stations": "Bierce Reach,Lubbock Platform,Baracchi Mines"
+ },
+ {
+ "name": "Mayoruband",
+ "pos_x": -73.84375,
+ "pos_y": -43.84375,
+ "pos_z": 102.1875,
+ "stations": "Atkov Beacon,Klimuk Terminal"
+ },
+ {
+ "name": "Mazahuanses",
+ "pos_x": 59.4375,
+ "pos_y": -24.28125,
+ "pos_z": 17,
+ "stations": "Messerschmid Orbital,Boltzmann Hub,Ramsbottom Hub"
+ },
+ {
+ "name": "Mazahun",
+ "pos_x": 90.84375,
+ "pos_y": -42.84375,
+ "pos_z": 25.03125,
+ "stations": "Arnold Dock"
+ },
+ {
+ "name": "Mazu",
+ "pos_x": -13.5625,
+ "pos_y": -71.59375,
+ "pos_z": -28.28125,
+ "stations": "Jacquard Terminal,Hernandez Dock,Manakov Hub,Ivanov Ring,Somerset Enterprise,Arkwright Hub,Yurchikhin Beacon,Cixin Mines,Kopra City,Yegorov Port,Windt Arsenal,Eisele Terminal"
+ },
+ {
+ "name": "Mbamba",
+ "pos_x": -82.0625,
+ "pos_y": -36.28125,
+ "pos_z": 135.375,
+ "stations": "Nikitin Enterprise,Priestley Dock,Ride Works"
+ },
+ {
+ "name": "Mbambiva",
+ "pos_x": 79.6875,
+ "pos_y": 20.875,
+ "pos_z": 24.25,
+ "stations": "Murray Terminal,Komarov Survey,Gillekens Platform,Boas Station,Starzl Refinery"
+ },
+ {
+ "name": "Mbamunians",
+ "pos_x": 147.03125,
+ "pos_y": -5.34375,
+ "pos_z": 48.46875,
+ "stations": "Griffin Terminal,Barton Bastion"
+ },
+ {
+ "name": "Mban",
+ "pos_x": 93.40625,
+ "pos_y": -15.5625,
+ "pos_z": 45.4375,
+ "stations": "Salk's Claim,Volta Hub"
+ },
+ {
+ "name": "Mbay",
+ "pos_x": -54.71875,
+ "pos_y": -55.96875,
+ "pos_z": 61.34375,
+ "stations": "Patterson Dock,Zillig Works"
+ },
+ {
+ "name": "Mbayaksas",
+ "pos_x": 182.53125,
+ "pos_y": -147.625,
+ "pos_z": 61.1875,
+ "stations": "Hildebrandt Landing"
+ },
+ {
+ "name": "Mbayame",
+ "pos_x": 57.4375,
+ "pos_y": -151.875,
+ "pos_z": -130.59375,
+ "stations": "Darnielle Depot"
+ },
+ {
+ "name": "Mbayo",
+ "pos_x": -104.25,
+ "pos_y": -99.6875,
+ "pos_z": 22.8125,
+ "stations": "Hopkins Plant,Diesel Dock"
+ },
+ {
+ "name": "Mbaza",
+ "pos_x": 120.0625,
+ "pos_y": -120.03125,
+ "pos_z": 8.53125,
+ "stations": "Dolgov Vision,Homer Platform,Sikorsky Hub"
+ },
+ {
+ "name": "Mbazak",
+ "pos_x": -98.03125,
+ "pos_y": -58.71875,
+ "pos_z": -39.34375,
+ "stations": "Morgan Dock"
+ },
+ {
+ "name": "Mbegera",
+ "pos_x": -13.03125,
+ "pos_y": 169.875,
+ "pos_z": 31.96875,
+ "stations": "Dorsey Gateway,Malocello Station,Spielberg Terminal"
+ },
+ {
+ "name": "Mbegua",
+ "pos_x": 136.4375,
+ "pos_y": -133.3125,
+ "pos_z": 86.90625,
+ "stations": "Cummings Vision,Wirtanen Orbital,Ulloa Survey,Ahmed Terminal"
+ },
+ {
+ "name": "Mbeguenchu",
+ "pos_x": -44.28125,
+ "pos_y": 19.875,
+ "pos_z": -114.25,
+ "stations": "al-Khayyam Colony,Russo Dock,Pippin Base"
+ },
+ {
+ "name": "Mber",
+ "pos_x": 107.75,
+ "pos_y": -75.4375,
+ "pos_z": 45.34375,
+ "stations": "Dreyer Base"
+ },
+ {
+ "name": "Mbera",
+ "pos_x": -125.40625,
+ "pos_y": 11.40625,
+ "pos_z": 46.4375,
+ "stations": "Litke Orbital,Coulter Enterprise,Grimwood City,Boswell Holdings,Shelley Landing"
+ },
+ {
+ "name": "Mbere",
+ "pos_x": 13.3125,
+ "pos_y": -85.71875,
+ "pos_z": 79.96875,
+ "stations": "Suzuki Orbital,Chaffee Survey,Marques Horizons,Kludze Silo"
+ },
+ {
+ "name": "Mbetani",
+ "pos_x": -133.875,
+ "pos_y": 40.5625,
+ "pos_z": 88.5625,
+ "stations": "Issigonis Station,Helmholtz Dock,Komarov Ring"
+ },
+ {
+ "name": "Mbetatika",
+ "pos_x": 46.5,
+ "pos_y": -132.75,
+ "pos_z": 11.375,
+ "stations": "Langley Point"
+ },
+ {
+ "name": "Mbo",
+ "pos_x": 67.09375,
+ "pos_y": 91.09375,
+ "pos_z": 43.875,
+ "stations": "Weitz Port,Galilei Platform"
+ },
+ {
+ "name": "Mbokokumal",
+ "pos_x": 84.71875,
+ "pos_y": 29.90625,
+ "pos_z": -105.75,
+ "stations": "Fernandes Colony,Caidin Platform"
+ },
+ {
+ "name": "Mbokomoteno",
+ "pos_x": 34.65625,
+ "pos_y": 76.21875,
+ "pos_z": -125.9375,
+ "stations": "Komarov Settlement,Cockrell Colony,Dedekind Beacon"
+ },
+ {
+ "name": "Mbokos",
+ "pos_x": 101.09375,
+ "pos_y": -85.71875,
+ "pos_z": -66.34375,
+ "stations": "Cadamosto Dock,Froude Dock,Lichtenberg Arsenal,Sarmiento de Gamboa Terminal"
+ },
+ {
+ "name": "Mbola",
+ "pos_x": -17.1875,
+ "pos_y": -3.34375,
+ "pos_z": 136.21875,
+ "stations": "Fawcett Stop,Hiraga Port,Marlowe Base"
+ },
+ {
+ "name": "Mbolgargl",
+ "pos_x": -102.84375,
+ "pos_y": -118.15625,
+ "pos_z": 22.96875,
+ "stations": "Priest Landing,Tanaka Horizons,Elder Mine"
+ },
+ {
+ "name": "Mboyan",
+ "pos_x": -19.125,
+ "pos_y": -101.6875,
+ "pos_z": -30.78125,
+ "stations": "Chaudhary Hangar,Gaspar de Portola Holdings"
+ },
+ {
+ "name": "Mbukarla",
+ "pos_x": 49.09375,
+ "pos_y": 0.84375,
+ "pos_z": -82.5,
+ "stations": "Mitropoulos Arsenal,Gibson Lab,Willis Hangar,Meikle Hub"
+ },
+ {
+ "name": "Mbukas",
+ "pos_x": 106.75,
+ "pos_y": -118.625,
+ "pos_z": 109.28125,
+ "stations": "Schaumasse Hub,Jekhowsky Orbital,Hughes Port,Nagata Works,Stuart Silo"
+ },
+ {
+ "name": "Mbukuravi",
+ "pos_x": -31.875,
+ "pos_y": -13.125,
+ "pos_z": 57.34375,
+ "stations": "Musgrave Relay,Fullerton Horizons,Alten Settlement"
+ },
+ {
+ "name": "Mbukushu",
+ "pos_x": -58.375,
+ "pos_y": 52.3125,
+ "pos_z": 115.9375,
+ "stations": "He Penal colony,Guerrero Relay"
+ },
+ {
+ "name": "Mbundu",
+ "pos_x": -32.78125,
+ "pos_y": -60,
+ "pos_z": 129.03125,
+ "stations": "Kroehl Landing,Laing Terminal"
+ },
+ {
+ "name": "Mbungga",
+ "pos_x": 90.15625,
+ "pos_y": -154.90625,
+ "pos_z": -57.15625,
+ "stations": "Grijalva Relay,von Zach Terminal,Hale Plant"
+ },
+ {
+ "name": "Mbutas",
+ "pos_x": 27.71875,
+ "pos_y": -128.5,
+ "pos_z": -48.96875,
+ "stations": "Goulart Port,Dozois Terminal,Burkin Orbital,McDevitt Orbital,Chun Installation,Nicholson Holdings,Nearchus Installation,Darlton Port,Fernao do Po Enterprise,Converse Gateway,Fan Landing"
+ },
+ {
+ "name": "Mbutia",
+ "pos_x": 57.4375,
+ "pos_y": 106.625,
+ "pos_z": -12.0625,
+ "stations": "Baraniecki's Pride,Offutt Point,Holden Dock"
+ },
+ {
+ "name": "Mbuts",
+ "pos_x": -135.0625,
+ "pos_y": -71.625,
+ "pos_z": -60.53125,
+ "stations": "Hyecho Reach,Swift Legacy"
+ },
+ {
+ "name": "Mbutsi",
+ "pos_x": 50.59375,
+ "pos_y": 15.125,
+ "pos_z": -3.90625,
+ "stations": "Drake Settlement,Andrews Landing,Scortia Dock,Erikson Vision"
+ },
+ {
+ "name": "MCC 105",
+ "pos_x": -83,
+ "pos_y": 44.9375,
+ "pos_z": -67.15625,
+ "stations": "Beregovoi Hangar,Thomson Base,Bella Installation"
+ },
+ {
+ "name": "MCC 296",
+ "pos_x": -8.3125,
+ "pos_y": 115.8125,
+ "pos_z": -12.28125,
+ "stations": "Ziemkiewicz Vision,Bachman Prospect,Zahn Hub,Crowley Installation,Hodgson Holdings,Martinez Enterprise"
+ },
+ {
+ "name": "MCC 378",
+ "pos_x": -56.40625,
+ "pos_y": -27.59375,
+ "pos_z": -50.15625,
+ "stations": "Lasswitz Orbital,Pippin Port,Waldrop Installation"
+ },
+ {
+ "name": "MCC 445",
+ "pos_x": 5.5625,
+ "pos_y": -45.1875,
+ "pos_z": -102.5,
+ "stations": "Aksyonov Orbital,Bamford Enterprise,Tyson Landing,Wright's Folly,Gamow Terminal,Gaspar de Portola Arena,MacLeod Reach,Bulgarin's Folly,Avogadro Bastion"
+ },
+ {
+ "name": "MCC 460",
+ "pos_x": -61.53125,
+ "pos_y": 33.65625,
+ "pos_z": -95.28125,
+ "stations": "Friesner Gateway,Thompson City,Polya City,Massimino Legacy,Rodrigues Dock,Hunt Station,Christopher City,Merchiston Terminal,Bryant Station,Perry Landing,Barjavel Refinery,Kurland Reach,Matthews Landing,Huxley's Progress"
+ },
+ {
+ "name": "MCC 467",
+ "pos_x": 13.8125,
+ "pos_y": -8.71875,
+ "pos_z": -67.71875,
+ "stations": "Fuca Ring,Brunel Port,Hunt Base,Ron Hubbard Ring,Cook Penal colony"
+ },
+ {
+ "name": "MCC 549",
+ "pos_x": 7.96875,
+ "pos_y": 82.53125,
+ "pos_z": -72.40625,
+ "stations": "Pippin Dock,Biggle Dock,Wyndham Dock,Oefelein Depot"
+ },
+ {
+ "name": "MCC 561",
+ "pos_x": 26.90625,
+ "pos_y": 90,
+ "pos_z": -62.9375,
+ "stations": "Heck Mines"
+ },
+ {
+ "name": "MCC 572",
+ "pos_x": 41.1875,
+ "pos_y": 91.4375,
+ "pos_z": -51.59375,
+ "stations": "Levinson Keep,Sanger Terminal,Feoktistov Depot"
+ },
+ {
+ "name": "MCC 613",
+ "pos_x": 65.40625,
+ "pos_y": 90.65625,
+ "pos_z": -0.71875,
+ "stations": "Webb Dock,Tokubei Colony,Burnham Relay"
+ },
+ {
+ "name": "MCC 684",
+ "pos_x": -44.9375,
+ "pos_y": 93.4375,
+ "pos_z": -24.28125,
+ "stations": "Clement Orbital,Landsteiner City"
+ },
+ {
+ "name": "MCC 686",
+ "pos_x": 8.75,
+ "pos_y": 80.875,
+ "pos_z": 13.65625,
+ "stations": "Smith City,Baudin Station,Harrison Hub,Bisson Penal colony,Bester Prospect,Malzberg's Folly"
+ },
+ {
+ "name": "MCC 741",
+ "pos_x": -47.1875,
+ "pos_y": 94.40625,
+ "pos_z": 42.03125,
+ "stations": "Crown Hub,Sakers Port,Mitchison Enterprise"
+ },
+ {
+ "name": "MCC 818",
+ "pos_x": -78.96875,
+ "pos_y": 5.875,
+ "pos_z": 13.84375,
+ "stations": "Herreshoff Relay,Whitney Orbital,Hauck Orbital"
+ },
+ {
+ "name": "MCC 858",
+ "pos_x": -74,
+ "pos_y": 4.9375,
+ "pos_z": -29.875,
+ "stations": "Rennie City,Al-Farabi Port,Kondratyev Point,Nachtigal Horizons,Fraley Orbital"
+ },
+ {
+ "name": "MCC 868",
+ "pos_x": -63.78125,
+ "pos_y": -39.71875,
+ "pos_z": -21.15625,
+ "stations": "Swanson Port,Lucid Dock"
+ },
+ {
+ "name": "MCC 872",
+ "pos_x": -121.0625,
+ "pos_y": -63.3125,
+ "pos_z": -49.34375,
+ "stations": "Butz Terminal,Jendrassik Hub,Gerst Terminal"
+ },
+ {
+ "name": "Mdi",
+ "pos_x": -40.46875,
+ "pos_y": 12.28125,
+ "pos_z": -128.6875,
+ "stations": "Glenn Park,Viktorenko Platform,Sommerfeld Port"
+ },
+ {
+ "name": "Mebech",
+ "pos_x": 88.8125,
+ "pos_y": -3.0625,
+ "pos_z": -0.21875,
+ "stations": "Stuart Base,Ostwald Holdings,Bulgarin Depot,Howard Hub"
+ },
+ {
+ "name": "Mebech Tzu",
+ "pos_x": 58.65625,
+ "pos_y": 77.21875,
+ "pos_z": -61.46875,
+ "stations": "Cayley Platform,Vetulani Installation,Smith Plant"
+ },
+ {
+ "name": "Mebechtani",
+ "pos_x": 57.21875,
+ "pos_y": 0.03125,
+ "pos_z": 69.03125,
+ "stations": "Guin Prospect"
+ },
+ {
+ "name": "Mebegernir",
+ "pos_x": 50.6875,
+ "pos_y": -67.1875,
+ "pos_z": -12.8125,
+ "stations": "Cenker Orbital,Weber Station,McCormick Port,Hottot Palace"
+ },
+ {
+ "name": "Mebekre",
+ "pos_x": 18.96875,
+ "pos_y": -124.1875,
+ "pos_z": 34.21875,
+ "stations": "West Mines,Walter Terminal"
+ },
+ {
+ "name": "Mebes",
+ "pos_x": 86.90625,
+ "pos_y": -12.3125,
+ "pos_z": 71,
+ "stations": "Anderson Terminal,Kohoutek Gateway,Asaro Port,Herbig Dock"
+ },
+ {
+ "name": "Mech",
+ "pos_x": -80.78125,
+ "pos_y": 160.84375,
+ "pos_z": -39.375,
+ "stations": "Evangelisti Gateway,MacCurdy Hub,Nourse Port,Cowper's Claim,Clute's Progress"
+ },
+ {
+ "name": "Mechagii",
+ "pos_x": 26.09375,
+ "pos_y": 116.125,
+ "pos_z": 117.375,
+ "stations": "Bulgarin Landing,Herbert Platform,Ostwald Settlement"
+ },
+ {
+ "name": "Meche",
+ "pos_x": 37.875,
+ "pos_y": 38.53125,
+ "pos_z": 79.40625,
+ "stations": "Rontgen Enterprise,Cooper Relay,Ford Landing"
+ },
+ {
+ "name": "Mechehooit",
+ "pos_x": 87.59375,
+ "pos_y": -232,
+ "pos_z": 50.09375,
+ "stations": "Shirazi Arena,Rae Mines"
+ },
+ {
+ "name": "Mechelkanu",
+ "pos_x": -211.21875,
+ "pos_y": 21.15625,
+ "pos_z": -11,
+ "stations": "Leckie Arena,Krupkat Relay"
+ },
+ {
+ "name": "Mecheng",
+ "pos_x": 131.125,
+ "pos_y": -161.28125,
+ "pos_z": -16.125,
+ "stations": "Otomo's Claim,Shepherd Point,Evans' Claim"
+ },
+ {
+ "name": "Mechet",
+ "pos_x": -21.0625,
+ "pos_y": 40.46875,
+ "pos_z": 115.6875,
+ "stations": "Tito Base,Robson Port,Delany Reach,Crown Ring"
+ },
+ {
+ "name": "Mecht",
+ "pos_x": -84.84375,
+ "pos_y": -75.78125,
+ "pos_z": -12.4375,
+ "stations": "Stefanyshyn-Piper Installation,Thiele Point,Deb Relay"
+ },
+ {
+ "name": "Mechtan",
+ "pos_x": -95.5625,
+ "pos_y": -17.84375,
+ "pos_z": 62.21875,
+ "stations": "Kuttner Vision,Pike Depot"
+ },
+ {
+ "name": "Mechtanitou",
+ "pos_x": 122.78125,
+ "pos_y": -113.53125,
+ "pos_z": 37.375,
+ "stations": "Bharadwaj Terminal,Denning Landing,Milnor Penal colony,Reynolds Holdings"
+ },
+ {
+ "name": "Mechucos",
+ "pos_x": 67.03125,
+ "pos_y": 39.59375,
+ "pos_z": -70.09375,
+ "stations": "Fossum Hub,Brandenstein Port,Ochoa Arsenal,Treshchov Station,Gerlache Plant"
+ },
+ {
+ "name": "Medb",
+ "pos_x": 12.78125,
+ "pos_y": 4.8125,
+ "pos_z": 39.34375,
+ "stations": "Vela Dock,Clark Terminal,Meade Terminal,Lie Silo"
+ },
+ {
+ "name": "Medei",
+ "pos_x": 159.96875,
+ "pos_y": -198.71875,
+ "pos_z": -2.6875,
+ "stations": "Royo Outpost"
+ },
+ {
+ "name": "Medeina",
+ "pos_x": 82.03125,
+ "pos_y": -229.9375,
+ "pos_z": -37.875,
+ "stations": "Arisman Mines,Christy Holdings"
+ },
+ {
+ "name": "Medimunda",
+ "pos_x": 47.5625,
+ "pos_y": -33.78125,
+ "pos_z": 86.625,
+ "stations": "Killough Relay,Valdes City,Okorafor Terminal,Bernoulli Enterprise,Smith Hub,Whymper City,Charnas Holdings"
+ },
+ {
+ "name": "Medj He",
+ "pos_x": -83.625,
+ "pos_y": -33.4375,
+ "pos_z": 42.34375,
+ "stations": "Grissom Gateway"
+ },
+ {
+ "name": "Medu",
+ "pos_x": -1.6875,
+ "pos_y": 168.90625,
+ "pos_z": 61.0625,
+ "stations": "McArthur Hub"
+ },
+ {
+ "name": "Medui",
+ "pos_x": 82.9375,
+ "pos_y": -222.875,
+ "pos_z": 21.03125,
+ "stations": "Dowty Hub,Hansen Orbital,McKean Platform"
+ },
+ {
+ "name": "Medungni",
+ "pos_x": 42.40625,
+ "pos_y": -11.3125,
+ "pos_z": 131.59375,
+ "stations": "He Hub,Bester Enterprise,Jeschke Installation"
+ },
+ {
+ "name": "Medusa",
+ "pos_x": -67.96875,
+ "pos_y": 42.5,
+ "pos_z": -14.5,
+ "stations": "Kopra City"
+ },
+ {
+ "name": "Meduwang",
+ "pos_x": 118.9375,
+ "pos_y": -64.1875,
+ "pos_z": 32,
+ "stations": "Barnes Arsenal,Lloyd Wright Park,Leopold Heckmann Dock"
+ },
+ {
+ "name": "Medzihozo",
+ "pos_x": 162.4375,
+ "pos_y": -60.5625,
+ "pos_z": -9.625,
+ "stations": "Grunsfeld Relay,Williams' Folly"
+ },
+ {
+ "name": "Medziojin",
+ "pos_x": -15.375,
+ "pos_y": -100.5,
+ "pos_z": -80.78125,
+ "stations": "Cooper Settlement,Banks Terminal,Norton Base"
+ },
+ {
+ "name": "Medzist",
+ "pos_x": -39.03125,
+ "pos_y": -121.9375,
+ "pos_z": -104.6875,
+ "stations": "Abbott Orbital,Bernoulli Vision,Galouye Dock,Cayley Hub"
+ },
+ {
+ "name": "Medzistha",
+ "pos_x": -44.4375,
+ "pos_y": -69.6875,
+ "pos_z": 98.875,
+ "stations": "Cook Gateway"
+ },
+ {
+ "name": "Medzisti",
+ "pos_x": -87.34375,
+ "pos_y": -19.15625,
+ "pos_z": -59.5625,
+ "stations": "Turzillo Plant,Niven Prospect"
+ },
+ {
+ "name": "Medzistine",
+ "pos_x": 117.90625,
+ "pos_y": -11.3125,
+ "pos_z": 49.5,
+ "stations": "Galton Port,Hart Orbital,Lorrah Point"
+ },
+ {
+ "name": "Meelov",
+ "pos_x": 8.28125,
+ "pos_y": 55.03125,
+ "pos_z": -64.0625,
+ "stations": "So-yeon Relay,Poleshchuk Dock,Guidoni Laboratory"
+ },
+ {
+ "name": "Meenapati",
+ "pos_x": 16.28125,
+ "pos_y": -91.125,
+ "pos_z": 100.75,
+ "stations": "Herzog Terminal"
+ },
+ {
+ "name": "Meenas",
+ "pos_x": -112.9375,
+ "pos_y": 4.40625,
+ "pos_z": -71.21875,
+ "stations": "Bain Vision,Molina Hangar"
+ },
+ {
+ "name": "Meenates",
+ "pos_x": -78.71875,
+ "pos_y": 53.5625,
+ "pos_z": -23.4375,
+ "stations": "Glazkov Station,Burbank Station,Comino Station"
+ },
+ {
+ "name": "Meene",
+ "pos_x": 118.78125,
+ "pos_y": -56.4375,
+ "pos_z": -97.1875,
+ "stations": "Felice Dock,Phoenix Base,Mitchell Dock,Weber Dock,Schiltberger's Progress"
+ },
+ {
+ "name": "Meeneratii",
+ "pos_x": 141.34375,
+ "pos_y": -9.28125,
+ "pos_z": 10.15625,
+ "stations": "Ansari Dock,Buchli Terminal,Smith Orbital,Stefanyshyn-Piper Terminal,Klink Vision,Marconi Dock,Weitz Works,Ahmed's Folly,Busch Arsenal"
+ },
+ {
+ "name": "Megrez",
+ "pos_x": -30.09375,
+ "pos_y": 69.5625,
+ "pos_z": -27.1875,
+ "stations": "Godel Dock,Lanier Vision,Clark Terminal,Hudson Enterprise,Clement Terminal,Shepherd Palace,Yano Installation,Panshin Terminal"
+ },
+ {
+ "name": "Mehen",
+ "pos_x": -12.78125,
+ "pos_y": 57.96875,
+ "pos_z": 122.9375,
+ "stations": "Oersted Gateway,MacLean Port,Flynn Installation"
+ },
+ {
+ "name": "Mehet",
+ "pos_x": 33.71875,
+ "pos_y": 12.4375,
+ "pos_z": 72.5,
+ "stations": "Schwann Dock,Scott Station"
+ },
+ {
+ "name": "Mehime",
+ "pos_x": 43.625,
+ "pos_y": -80.0625,
+ "pos_z": 80.75,
+ "stations": "Bode Vision,Sugie Landing,Nelson Relay,Alphonsi Bastion,Dingle Survey"
+ },
+ {
+ "name": "Mehimurt",
+ "pos_x": -131.28125,
+ "pos_y": 99.90625,
+ "pos_z": -39.875,
+ "stations": "Pippin Terminal,Eisenstein Station,Bear Port,Stabenow Vista"
+ },
+ {
+ "name": "Mehinn",
+ "pos_x": 15.6875,
+ "pos_y": 160.8125,
+ "pos_z": -41.53125,
+ "stations": "Mallett Enterprise,Kirtley Sanctuary"
+ },
+ {
+ "name": "Mehit",
+ "pos_x": 24.53125,
+ "pos_y": 137.3125,
+ "pos_z": -36.375,
+ "stations": "Bottego Hub,Panshin Station,Pytheas' Folly,Hume Survey"
+ },
+ {
+ "name": "Mehita",
+ "pos_x": 70.5625,
+ "pos_y": 44.40625,
+ "pos_z": 144.28125,
+ "stations": "Heyerdahl Depot,Narvaez Arena"
+ },
+ {
+ "name": "Mehitak",
+ "pos_x": -123.09375,
+ "pos_y": 0.5,
+ "pos_z": 75.1875,
+ "stations": "Skolem Refinery,Vonnegut Works"
+ },
+ {
+ "name": "Mehnemeowa",
+ "pos_x": -119.03125,
+ "pos_y": -59.6875,
+ "pos_z": 101,
+ "stations": "Woolley Works,Celebi Survey,Campbell Enterprise"
+ },
+ {
+ "name": "Mehnemind",
+ "pos_x": 133.96875,
+ "pos_y": -201.40625,
+ "pos_z": -13.5,
+ "stations": "Flammarion Hub,Parsons Reach,Tombaugh Orbital"
+ },
+ {
+ "name": "Mehnemine",
+ "pos_x": 77.78125,
+ "pos_y": 42.5,
+ "pos_z": -35.34375,
+ "stations": "Meaney Base,Remek Port"
+ },
+ {
+ "name": "Mehua",
+ "pos_x": -134.71875,
+ "pos_y": 24.46875,
+ "pos_z": -57.5625,
+ "stations": "Payette Enterprise,Volkov Hub,Virtanen Ring,Perry Vista"
+ },
+ {
+ "name": "Mehudi",
+ "pos_x": 68.59375,
+ "pos_y": -20.59375,
+ "pos_z": -31.0625,
+ "stations": "Reisman Station,Melvin's Inheritance,Ivins Ring,Dana Station"
+ },
+ {
+ "name": "Mehudti",
+ "pos_x": -12.46875,
+ "pos_y": -99.28125,
+ "pos_z": -20.9375,
+ "stations": "Pawelczyk Station"
+ },
+ {
+ "name": "Mehuenomici",
+ "pos_x": 83.28125,
+ "pos_y": -81.28125,
+ "pos_z": 56.78125,
+ "stations": "Gorey Ring,Bohr Gateway,Kaneda Orbital,Oberth Depot"
+ },
+ {
+ "name": "Mehurs",
+ "pos_x": -95.96875,
+ "pos_y": -74.0625,
+ "pos_z": 59.84375,
+ "stations": "McDevitt Station,Schroeder Dock,Lynn Vision,Lebesgue Dock,Grimwood Station,Lobachevsky Enterprise,Celebi Prospect"
+ },
+ {
+ "name": "Meidjing",
+ "pos_x": 37.84375,
+ "pos_y": -93.125,
+ "pos_z": -74.21875,
+ "stations": "Daqing Gateway,Herodotus Prospect,Hynek Station"
+ },
+ {
+ "name": "Meidmarama",
+ "pos_x": -120.28125,
+ "pos_y": 61.8125,
+ "pos_z": 109.28125,
+ "stations": "Back Port,Nielsen Dock,Eisenstein Works"
+ },
+ {
+ "name": "Meidruwa",
+ "pos_x": -139.9375,
+ "pos_y": -21.21875,
+ "pos_z": 101.90625,
+ "stations": "Jeury Arena"
+ },
+ {
+ "name": "Meidruwutii",
+ "pos_x": 83.75,
+ "pos_y": -221.5,
+ "pos_z": 57.1875,
+ "stations": "Lindemann Outpost,Charlois Depot"
+ },
+ {
+ "name": "Meidubi",
+ "pos_x": -2.21875,
+ "pos_y": -8.84375,
+ "pos_z": 76.6875,
+ "stations": "Dzhanibekov Ring,Frimout Port,Carson Orbital,Mayer Orbital,Sullivan Dock,Leavitt Hub,Arkwright City,Metcalf City,Jenner Orbital,Chasles Depot,Rukavishnikov's Inheritance"
+ },
+ {
+ "name": "Meinannovi",
+ "pos_x": -89.65625,
+ "pos_y": -80.6875,
+ "pos_z": -1.8125,
+ "stations": "Tryggvason Observatory,Mieville Dock"
+ },
+ {
+ "name": "Meinjhalara",
+ "pos_x": -66.375,
+ "pos_y": 73.6875,
+ "pos_z": -16.5625,
+ "stations": "Fremont Port,Forsskal Ring"
+ },
+ {
+ "name": "Meinjhalie",
+ "pos_x": -140.65625,
+ "pos_y": 44.8125,
+ "pos_z": -64.53125,
+ "stations": "Vasyutin's Claim,McCandless Base"
+ },
+ {
+ "name": "Meintangk",
+ "pos_x": 44.75,
+ "pos_y": -14.75,
+ "pos_z": 121.5625,
+ "stations": "Struve Works"
+ },
+ {
+ "name": "Meiri",
+ "pos_x": -28.03125,
+ "pos_y": -85.15625,
+ "pos_z": -37.34375,
+ "stations": "Terry Survey,Grunsfeld Dock,Borman Platform,Tognini Dock"
+ },
+ {
+ "name": "Mel 22 Sector GM-V c2-8",
+ "pos_x": -192.125,
+ "pos_y": -194.96875,
+ "pos_z": -471.65625,
+ "stations": "Viridian Orbital"
+ },
+ {
+ "name": "Melchei",
+ "pos_x": 82.21875,
+ "pos_y": 49.53125,
+ "pos_z": -99.40625,
+ "stations": "Hassanein Colony"
+ },
+ {
+ "name": "Melcior",
+ "pos_x": 54.78125,
+ "pos_y": 65.25,
+ "pos_z": -9.21875,
+ "stations": "Belyayev Landing,Low Hub"
+ },
+ {
+ "name": "Meldrith",
+ "pos_x": 25.21875,
+ "pos_y": 68.75,
+ "pos_z": 40.46875,
+ "stations": "Wilson Station,Janszoon Depot,Cayley Legacy"
+ },
+ {
+ "name": "Meli",
+ "pos_x": 39.375,
+ "pos_y": 89.84375,
+ "pos_z": -112,
+ "stations": "Haise Beacon"
+ },
+ {
+ "name": "Meliae",
+ "pos_x": -17.3125,
+ "pos_y": 49.53125,
+ "pos_z": -1.6875,
+ "stations": "Whitson Hub,Hadfield Hub,Carr Terminal"
+ },
+ {
+ "name": "Melici",
+ "pos_x": 89.6875,
+ "pos_y": -1.8125,
+ "pos_z": -33,
+ "stations": "Alpers Bastion,Yeliseyev Settlement,Polansky Landing"
+ },
+ {
+ "name": "Melingo",
+ "pos_x": -165.78125,
+ "pos_y": -33.34375,
+ "pos_z": 0.375,
+ "stations": "Dias' Claim"
+ },
+ {
+ "name": "Melingoi",
+ "pos_x": 80.9375,
+ "pos_y": -47.21875,
+ "pos_z": -134.59375,
+ "stations": "Hale Camp"
+ },
+ {
+ "name": "Melinoe",
+ "pos_x": -89.375,
+ "pos_y": -38.65625,
+ "pos_z": 55.0625,
+ "stations": "Hand Terminal,McAllaster Landing"
+ },
+ {
+ "name": "Meliontit",
+ "pos_x": 67.34375,
+ "pos_y": -136.625,
+ "pos_z": -2.84375,
+ "stations": "Abe Hub,Littrow Ring,Dawson City,Calatrava Arsenal,Powell Terminal"
+ },
+ {
+ "name": "Meminii",
+ "pos_x": -3.03125,
+ "pos_y": 64.78125,
+ "pos_z": -51,
+ "stations": "White Platform,Lowry Terminal,Fadlan Settlement"
+ },
+ {
+ "name": "Men Samit",
+ "pos_x": -122.9375,
+ "pos_y": 63.875,
+ "pos_z": -36.03125,
+ "stations": "Redi Camp,Tarelkin Settlement,Szebehely Colony"
+ },
+ {
+ "name": "Men Shen",
+ "pos_x": 35.125,
+ "pos_y": 9.53125,
+ "pos_z": 67.03125,
+ "stations": "Shukor Dock,Solovyov Terminal,Littlewood Relay"
+ },
+ {
+ "name": "Men Shi",
+ "pos_x": -82.125,
+ "pos_y": -1.40625,
+ "pos_z": 79.9375,
+ "stations": "Harper Dock"
+ },
+ {
+ "name": "Menambe",
+ "pos_x": -60,
+ "pos_y": 10.96875,
+ "pos_z": 57.1875,
+ "stations": "Beadle Settlement,Fourier Penal colony"
+ },
+ {
+ "name": "Menas",
+ "pos_x": 116.6875,
+ "pos_y": 87.125,
+ "pos_z": 23.375,
+ "stations": "Favier Settlement,Grunsfeld Landing,Poisson Refinery,Martinez Works"
+ },
+ {
+ "name": "Mendagorot",
+ "pos_x": 126.40625,
+ "pos_y": -206.125,
+ "pos_z": 21.09375,
+ "stations": "Tietjen Escape"
+ },
+ {
+ "name": "Mendego",
+ "pos_x": -102.9375,
+ "pos_y": 59.5,
+ "pos_z": 56.03125,
+ "stations": "Komarov City,Koch City,Davy Platform,Land Gateway,Herreshoff Works"
+ },
+ {
+ "name": "Mendindui",
+ "pos_x": 98.875,
+ "pos_y": 14.71875,
+ "pos_z": -25.0625,
+ "stations": "Priestley Terminal,Cernan City,McArthur Base,Crown Horizons"
+ },
+ {
+ "name": "Meneratunu",
+ "pos_x": -124.28125,
+ "pos_y": -82.34375,
+ "pos_z": -88.25,
+ "stations": "Flint Orbital,Euthymenes Laboratory,Szentmartony City,Bester Base"
+ },
+ {
+ "name": "Menero Kimi",
+ "pos_x": 79.125,
+ "pos_y": -204.8125,
+ "pos_z": -40.78125,
+ "stations": "Reichelt Camp"
+ },
+ {
+ "name": "Menes",
+ "pos_x": 74.25,
+ "pos_y": -35.75,
+ "pos_z": 48.40625,
+ "stations": "Bethe Holdings,Rzeppa Landing,Kotov Escape"
+ },
+ {
+ "name": "Meng",
+ "pos_x": 174.71875,
+ "pos_y": -115.5,
+ "pos_z": 25,
+ "stations": "Rizvi Platform"
+ },
+ {
+ "name": "Meng Mu",
+ "pos_x": -44.40625,
+ "pos_y": -3.46875,
+ "pos_z": 141.71875,
+ "stations": "Crowley Prospect,Lorrah Settlement,Shirley Depot,Rubruck Installation"
+ },
+ {
+ "name": "Menguru",
+ "pos_x": 72.3125,
+ "pos_y": -73.40625,
+ "pos_z": 103.46875,
+ "stations": "Reamy Relay,William Sargent Orbital,Schoening Orbital,Parkinson Ring,Lorrah's Folly,McMullen Lab"
+ },
+ {
+ "name": "Menhitae",
+ "pos_x": -80.5625,
+ "pos_y": 78.9375,
+ "pos_z": -141.625,
+ "stations": "Lebesgue Vision,Sauma Prospect,Coles Relay"
+ },
+ {
+ "name": "Menhures",
+ "pos_x": -75.40625,
+ "pos_y": -96.53125,
+ "pos_z": -36.65625,
+ "stations": "Morey's Claim"
+ },
+ {
+ "name": "Menkent",
+ "pos_x": 34.625,
+ "pos_y": 23.59375,
+ "pos_z": 41.25,
+ "stations": "Cenker Dock,Hamilton Lab,Porsche Works"
+ },
+ {
+ "name": "Mentesuri",
+ "pos_x": 117.53125,
+ "pos_y": 18.03125,
+ "pos_z": -96.4375,
+ "stations": "Snyder Enterprise,Aubakirov Terminal,Chaudhary Enterprise,Coulter Vision,Kagan Prospect"
+ },
+ {
+ "name": "Mentha",
+ "pos_x": 49.96875,
+ "pos_y": -109.59375,
+ "pos_z": 123.21875,
+ "stations": "Wilhelm Survey,Sweet Dock,Elwood Base"
+ },
+ {
+ "name": "Mentiens",
+ "pos_x": -32.28125,
+ "pos_y": 21.9375,
+ "pos_z": 81.65625,
+ "stations": "Ryman Port,Stasheff Enterprise,Foden Orbital,McKay Enterprise,Hassanein Survey"
+ },
+ {
+ "name": "Mentintei",
+ "pos_x": 47.03125,
+ "pos_y": -147.6875,
+ "pos_z": -29.34375,
+ "stations": "Blaauw Platform,Giraud Camp"
+ },
+ {
+ "name": "Mentor",
+ "pos_x": -27.65625,
+ "pos_y": -3.5,
+ "pos_z": -85.53125,
+ "stations": "Wnuk-Lipinski Observatory,Lasswitz Terminal,Acharya Station,Wood Relay"
+ },
+ {
+ "name": "Mentri",
+ "pos_x": 183.09375,
+ "pos_y": -122.875,
+ "pos_z": 2.21875,
+ "stations": "Fowler Mine"
+ },
+ {
+ "name": "Mentsuchua",
+ "pos_x": 96.96875,
+ "pos_y": -62.78125,
+ "pos_z": 20.84375,
+ "stations": "Carstensz Base,Hulse Holdings"
+ },
+ {
+ "name": "Menusha",
+ "pos_x": 127.53125,
+ "pos_y": -106.28125,
+ "pos_z": 61.78125,
+ "stations": "Seitter Orbital,Brill Terminal,Kobayashi Ring,Ryle Ring,Gabrielli Ring,Truman Gateway,Mohr Vision,Niijima Survey,Hoyle Horizons,Qureshi City,Alexandria Station,Slade Terminal"
+ },
+ {
+ "name": "Menutes",
+ "pos_x": -104.34375,
+ "pos_y": 82.4375,
+ "pos_z": 100.40625,
+ "stations": "Akiyama Gateway,Bernoulli Dock,Scully-Power Dock"
+ },
+ {
+ "name": "Merak",
+ "pos_x": -23.40625,
+ "pos_y": 65.53125,
+ "pos_z": -38.9375,
+ "stations": "Bottego Installation,Weyn Base,Eudoxus Beacon"
+ },
+ {
+ "name": "Mere",
+ "pos_x": 87.3125,
+ "pos_y": 62.28125,
+ "pos_z": -57.96875,
+ "stations": "Stott Vision,Bosch Station,Dobrovolskiy Dock"
+ },
+ {
+ "name": "Mereboga",
+ "pos_x": -55.8125,
+ "pos_y": 100.03125,
+ "pos_z": 9.09375,
+ "stations": "Howard Dock,Stackpole Landing,Kandel Barracks"
+ },
+ {
+ "name": "Merek",
+ "pos_x": 71.03125,
+ "pos_y": 8.875,
+ "pos_z": -130.4375,
+ "stations": "Qian Platform,Cayley Enterprise,Clark Platform"
+ },
+ {
+ "name": "Merengila",
+ "pos_x": 76.15625,
+ "pos_y": 113.40625,
+ "pos_z": 116.53125,
+ "stations": "Land's Exile,Bear Camp,Kessel's Inheritance"
+ },
+ {
+ "name": "Meretrida",
+ "pos_x": -9515.71875,
+ "pos_y": -899.625,
+ "pos_z": 19830.3125,
+ "stations": "Dezhnev Landing"
+ },
+ {
+ "name": "Meretseger",
+ "pos_x": 36.15625,
+ "pos_y": -24.65625,
+ "pos_z": -120.5625,
+ "stations": "Capek Terminal,Darlton City,Fiennes Hub,Forward Terminal,al-Haytham Plant,Dunyach City"
+ },
+ {
+ "name": "Meri",
+ "pos_x": -10.8125,
+ "pos_y": -25.40625,
+ "pos_z": -44.40625,
+ "stations": "Tyurin Port"
+ },
+ {
+ "name": "Merki",
+ "pos_x": -81.625,
+ "pos_y": 50.625,
+ "pos_z": -19.28125,
+ "stations": "Conti Hub,Delany Installation"
+ },
+ {
+ "name": "Merope",
+ "pos_x": -78.59375,
+ "pos_y": -149.625,
+ "pos_z": -340.53125,
+ "stations": "Alcazar's Hope,Omega Prospect,Reed's Rest,Rescue Ship - Reed's Rest"
+ },
+ {
+ "name": "Meropis",
+ "pos_x": 56.90625,
+ "pos_y": -133.59375,
+ "pos_z": 56.34375,
+ "stations": "Joseph Delambre Silo,Tlaloc Station,Laura Elizabeth,Cayley Landing"
+ },
+ {
+ "name": "Merti",
+ "pos_x": 42.03125,
+ "pos_y": 47.625,
+ "pos_z": 43.8125,
+ "stations": "Dorsey Prospect,McKay Silo,Coles Refinery"
+ },
+ {
+ "name": "Meru Kwarid",
+ "pos_x": 169.25,
+ "pos_y": -90.875,
+ "pos_z": 96.96875,
+ "stations": "Marcy Horizons,Mikoyan Landing,Schwarzschild Colony"
+ },
+ {
+ "name": "Merungas",
+ "pos_x": 4.46875,
+ "pos_y": 97.5625,
+ "pos_z": 98.0625,
+ "stations": "Fearn Horizons,Vancouver Station,Kotzebue Laboratory,Flinders Station,Webb Hub"
+ },
+ {
+ "name": "Meskhenga",
+ "pos_x": -53.28125,
+ "pos_y": -8.09375,
+ "pos_z": 110.5,
+ "stations": "Ham Port,Richards Terminal"
+ },
+ {
+ "name": "Meskhent",
+ "pos_x": 165.4375,
+ "pos_y": -164.96875,
+ "pos_z": -5.25,
+ "stations": "Engle Station,Johri Enterprise,Malvern Orbital,Cramer Vista"
+ },
+ {
+ "name": "Messa",
+ "pos_x": 62.5,
+ "pos_y": 47.6875,
+ "pos_z": -63.59375,
+ "stations": "Raleigh Landing,Polya Installation"
+ },
+ {
+ "name": "Messi",
+ "pos_x": -64.375,
+ "pos_y": 156.15625,
+ "pos_z": -19.71875,
+ "stations": "Quimper Vision,McCarthy Gateway,Lloyd Point,Watt-Evans Orbital,Powers Terminal"
+ },
+ {
+ "name": "MET 20",
+ "pos_x": -34.40625,
+ "pos_y": -55.5,
+ "pos_z": -89.75,
+ "stations": "Brady Ring"
+ },
+ {
+ "name": "Meticuli",
+ "pos_x": -77.09375,
+ "pos_y": 1.28125,
+ "pos_z": 37.15625,
+ "stations": "Mendeleev Point,McCulley Gateway"
+ },
+ {
+ "name": "Metzili",
+ "pos_x": -7.65625,
+ "pos_y": -46.8125,
+ "pos_z": -56.65625,
+ "stations": "Shepard Port,Ings Hub,Pippin Gateway,Makarov City,Shaw Station,Foster Depot,Zamyatin Gateway,Camarda Hub,Crichton Works,Boscovich Settlement,Grothendieck City,Swanwick Port"
+ },
+ {
+ "name": "Metztli",
+ "pos_x": -9518.40625,
+ "pos_y": -911.96875,
+ "pos_z": 19823.625,
+ "stations": "Vitto Orbital"
+ },
+ {
+ "name": "Mexicassa",
+ "pos_x": -41.09375,
+ "pos_y": -22.53125,
+ "pos_z": -151.90625,
+ "stations": "Mayr Terminal,Al-Jazari Vision"
+ },
+ {
+ "name": "Mexicatese",
+ "pos_x": 55.46875,
+ "pos_y": 41.84375,
+ "pos_z": -38.1875,
+ "stations": "Dobrovolski Station,Birkhoff Relay,Anthony's Progress,Casper Relay"
+ },
+ {
+ "name": "Mexicatuci",
+ "pos_x": 158.90625,
+ "pos_y": -145.65625,
+ "pos_z": 134.1875,
+ "stations": "Sternbach Colony"
+ },
+ {
+ "name": "Meza",
+ "pos_x": 37.4375,
+ "pos_y": -21.25,
+ "pos_z": -115.5,
+ "stations": "Andersson City,Klein Hangar"
+ },
+ {
+ "name": "Meza Virs",
+ "pos_x": -102.28125,
+ "pos_y": 30.1875,
+ "pos_z": 65.90625,
+ "stations": "Aldrin Vision,Sullivan Settlement"
+ },
+ {
+ "name": "Mezanik",
+ "pos_x": 24.15625,
+ "pos_y": -148.71875,
+ "pos_z": -79.0625,
+ "stations": "Flagg Dock,Carpenter Station,Gaughan Penal colony,Johnson Dock,Gardner Camp"
+ },
+ {
+ "name": "Mezaviates",
+ "pos_x": -135.125,
+ "pos_y": -38.25,
+ "pos_z": 74.625,
+ "stations": "Rond d'Alembert Terminal,Noether Bastion"
+ },
+ {
+ "name": "Mezavini",
+ "pos_x": -82.0625,
+ "pos_y": -20.75,
+ "pos_z": -36.375,
+ "stations": "Sellers Depot,Alexander Survey,Borman Works"
+ },
+ {
+ "name": "Mi He",
+ "pos_x": 0.71875,
+ "pos_y": -176.625,
+ "pos_z": 102.875,
+ "stations": "Spurzem Depot"
+ },
+ {
+ "name": "Mi Hsien",
+ "pos_x": -126.6875,
+ "pos_y": 119,
+ "pos_z": -3.78125,
+ "stations": "Conti Mine,Dall Laboratory"
+ },
+ {
+ "name": "Mi Hsing Wu",
+ "pos_x": 18.1875,
+ "pos_y": 2.21875,
+ "pos_z": 172.46875,
+ "stations": "Csoma Platform,Goulart Terminal,Vance Laboratory"
+ },
+ {
+ "name": "Mi Linkampi",
+ "pos_x": -15.125,
+ "pos_y": 91.4375,
+ "pos_z": -34.4375,
+ "stations": "Williams City,Bates Vision"
+ },
+ {
+ "name": "Mianomama",
+ "pos_x": -105.125,
+ "pos_y": -24.28125,
+ "pos_z": 106.15625,
+ "stations": "Musabayev Outpost,Moran Settlement,Velazquez Platform"
+ },
+ {
+ "name": "Miansis",
+ "pos_x": 131.78125,
+ "pos_y": -113.3125,
+ "pos_z": 120.5625,
+ "stations": "Carpenter Dock,Argelander Ring,Clute Base,Kulin Prospect,Godel Penal colony"
+ },
+ {
+ "name": "Miao Thixo",
+ "pos_x": -22.90625,
+ "pos_y": -21.375,
+ "pos_z": 81.71875,
+ "stations": "McDaniel Point,Plante Enterprise"
+ },
+ {
+ "name": "Miao Wang",
+ "pos_x": 90.03125,
+ "pos_y": -115.78125,
+ "pos_z": 128.25,
+ "stations": "Lewis Reach"
+ },
+ {
+ "name": "Michael Pantazis",
+ "pos_x": -32.59375,
+ "pos_y": -42.15625,
+ "pos_z": -65.65625,
+ "stations": "Due Colony,The Anna Marie Pantazis,Xuanzang Prospect,Drake Hub"
+ },
+ {
+ "name": "Michel",
+ "pos_x": 16.875,
+ "pos_y": -219.84375,
+ "pos_z": 56.9375,
+ "stations": "Witt Station,Vuia Station,Shute Orbital"
+ },
+ {
+ "name": "Mictlan",
+ "pos_x": 49.34375,
+ "pos_y": -93.9375,
+ "pos_z": 2.375,
+ "stations": "Kowal Dock,Myasishchev Orbital,South Hub,Sarmiento de Gamboa Plant"
+ },
+ {
+ "name": "Midgard",
+ "pos_x": -98.1875,
+ "pos_y": -11.46875,
+ "pos_z": -26.75,
+ "stations": "Rontgen Port"
+ },
+ {
+ "name": "Midgcut",
+ "pos_x": -14.625,
+ "pos_y": 10.34375,
+ "pos_z": 13.15625,
+ "stations": "Metcalf Dock,Piserchia Beacon"
+ },
+ {
+ "name": "Midi",
+ "pos_x": 104.5625,
+ "pos_y": -169.71875,
+ "pos_z": -38.0625,
+ "stations": "Wigura City,Alfven Orbital,Fraknoi Camp,Curtis Relay"
+ },
+ {
+ "name": "Mike",
+ "pos_x": -12.25,
+ "pos_y": -130.71875,
+ "pos_z": -10.84375,
+ "stations": "Kier Terminal,Nilson Port,Riazuddin Dock,Oshima City,Bereznyak's Inheritance"
+ },
+ {
+ "name": "Miki",
+ "pos_x": -111.21875,
+ "pos_y": -0.0625,
+ "pos_z": -46.71875,
+ "stations": "Coleman Dock,Garnier Lab"
+ },
+ {
+ "name": "Mikinii",
+ "pos_x": -17.5625,
+ "pos_y": -56.5625,
+ "pos_z": 133.9375,
+ "stations": "Shiras Beacon,Siodmak Beacon,Chilton Vision"
+ },
+ {
+ "name": "Mikinn",
+ "pos_x": -51.5625,
+ "pos_y": -26.0625,
+ "pos_z": 59.4375,
+ "stations": "Wallace Dock,Potagos Port,Heisenberg Reach"
+ },
+ {
+ "name": "Mikir",
+ "pos_x": 42.375,
+ "pos_y": -3.53125,
+ "pos_z": 0.5625,
+ "stations": "Alexander Beacon,Wang Hub,Shepard Hangar,Payette Settlement,Harrison's Cradle"
+ },
+ {
+ "name": "Miku",
+ "pos_x": -25.78125,
+ "pos_y": -11.25,
+ "pos_z": 158.71875,
+ "stations": "Younghusband Station,Schade Survey,Vinge City,Cowper Installation,Coles Settlement"
+ },
+ {
+ "name": "Mikunn",
+ "pos_x": -42.28125,
+ "pos_y": -51.78125,
+ "pos_z": 163.8125,
+ "stations": "Spassky's Inheritance"
+ },
+ {
+ "name": "Mikur",
+ "pos_x": 81.71875,
+ "pos_y": 71.5,
+ "pos_z": -53.53125,
+ "stations": "Kwolek Camp,Wescott's Claim"
+ },
+ {
+ "name": "Mikuylka",
+ "pos_x": 108.75,
+ "pos_y": 75.03125,
+ "pos_z": -91.34375,
+ "stations": "Knapp Dock"
+ },
+ {
+ "name": "Milatuknari",
+ "pos_x": 156.28125,
+ "pos_y": -80.9375,
+ "pos_z": 28.46875,
+ "stations": "Swanwick Holdings,Pons Vision,Hughes Port,Tall Settlement"
+ },
+ {
+ "name": "Milceni",
+ "pos_x": 103.375,
+ "pos_y": 14.625,
+ "pos_z": 15,
+ "stations": "Knipling Horizons,Wang Refinery,Langford Enterprise"
+ },
+ {
+ "name": "Milcenu",
+ "pos_x": -83.96875,
+ "pos_y": -103.375,
+ "pos_z": -19.46875,
+ "stations": "Berners-Lee Landing,Francisco de Eliza Penal colony,Aristotle Port"
+ },
+ {
+ "name": "Mildanji",
+ "pos_x": 131.125,
+ "pos_y": 77.78125,
+ "pos_z": -97.78125,
+ "stations": "Bruce Ring,Dummer Dock,Robinson Enterprise,MacLean Hub"
+ },
+ {
+ "name": "Mildeptu",
+ "pos_x": 14.125,
+ "pos_y": 1.3125,
+ "pos_z": -3.28125,
+ "stations": "Buchli City,Singer Enterprise,Wheelock Terminal"
+ },
+ {
+ "name": "Mile",
+ "pos_x": -82.90625,
+ "pos_y": 32.1875,
+ "pos_z": 112.09375,
+ "stations": "Sheffield Dock,Fisk Survey,Houtman Installation"
+ },
+ {
+ "name": "Mileke",
+ "pos_x": -124.71875,
+ "pos_y": -83.4375,
+ "pos_z": 97.21875,
+ "stations": "Jenkinson Settlement"
+ },
+ {
+ "name": "Mileku",
+ "pos_x": -107.53125,
+ "pos_y": 17.46875,
+ "pos_z": -72.96875,
+ "stations": "Hoffman Terminal,Dias Installation"
+ },
+ {
+ "name": "Milela",
+ "pos_x": 6.15625,
+ "pos_y": -43.59375,
+ "pos_z": 101.21875,
+ "stations": "Pailes Point,Baird Beacon"
+ },
+ {
+ "name": "Milelbis",
+ "pos_x": -75.8125,
+ "pos_y": 110.03125,
+ "pos_z": -59.75,
+ "stations": "Quick Dock,Helms Depot,Ray Dock,Stevin Station,Velho Station,Carrasco Port,Grover Installation"
+ },
+ {
+ "name": "Mill",
+ "pos_x": 122.75,
+ "pos_y": -34.90625,
+ "pos_z": 24.59375,
+ "stations": "Elson Relay"
+ },
+ {
+ "name": "Millcayac",
+ "pos_x": -21.4375,
+ "pos_y": -20.5625,
+ "pos_z": 169.90625,
+ "stations": "Berliner Platform,Smith Colony,Oramus Arsenal"
+ },
+ {
+ "name": "Miller",
+ "pos_x": -90.125,
+ "pos_y": 115.15625,
+ "pos_z": -22.875,
+ "stations": "McCaffrey Freeport,McAllaster Platform,Fourier Base"
+ },
+ {
+ "name": "Millese",
+ "pos_x": 15.25,
+ "pos_y": 20.25,
+ "pos_z": 56.03125,
+ "stations": "McMullen Ring,Lee Gateway,Janes Camp,Resnik Base"
+ },
+ {
+ "name": "Milpiriyo",
+ "pos_x": 66.5625,
+ "pos_y": -39.25,
+ "pos_z": -37.125,
+ "stations": "Willis Platform,Narvaez Survey"
+ },
+ {
+ "name": "Milscothach",
+ "pos_x": -9.59375,
+ "pos_y": -5.5,
+ "pos_z": 41.21875,
+ "stations": "Hennepin Penal colony,White Beacon"
+ },
+ {
+ "name": "Mimis",
+ "pos_x": 60.09375,
+ "pos_y": 15.375,
+ "pos_z": -96.15625,
+ "stations": "Helms Refinery,Vasyutin City"
+ },
+ {
+ "name": "Mimisina",
+ "pos_x": 155.5,
+ "pos_y": -194.71875,
+ "pos_z": 0.0625,
+ "stations": "Daqing Gateway,Walotsky Station,Ziewe Port,Gaspar de Portola Enterprise,Kratman Horizons"
+ },
+ {
+ "name": "Mimui",
+ "pos_x": -4,
+ "pos_y": -172.125,
+ "pos_z": 48,
+ "stations": "Wafa Enterprise,Edwards Hub,South's Folly"
+ },
+ {
+ "name": "Mimurtudji",
+ "pos_x": 50.53125,
+ "pos_y": 123.15625,
+ "pos_z": 24.75,
+ "stations": "Brooks Orbital,Rodrigues Dock,Russell City,Hodgson City,Hawke Hub,Pook City,Rushd Point,Heck's Pride"
+ },
+ {
+ "name": "Mimuruaye",
+ "pos_x": -100.5,
+ "pos_y": -100.9375,
+ "pos_z": 98.125,
+ "stations": "Bosch Orbital,Garneau Stop"
+ },
+ {
+ "name": "Mimuruin",
+ "pos_x": 75.90625,
+ "pos_y": 112.0625,
+ "pos_z": 59.125,
+ "stations": "Ochoa Orbital,Veach Terminal,Cori Plant,Lamarck Port,Darwin Keep"
+ },
+ {
+ "name": "Mimuthi",
+ "pos_x": -156,
+ "pos_y": -21.375,
+ "pos_z": -43.25,
+ "stations": "Forward Depot,Milnor Dock"
+ },
+ {
+ "name": "Mimutin",
+ "pos_x": -60.3125,
+ "pos_y": -57.59375,
+ "pos_z": 141.9375,
+ "stations": "Kiernan Holdings,Wallace Platform,Barreiros Holdings"
+ },
+ {
+ "name": "Minagnaiwa",
+ "pos_x": 59.5,
+ "pos_y": 35.1875,
+ "pos_z": -99.65625,
+ "stations": "Fettman Terminal,Dana's Inheritance,Torricelli Settlement,Revin Colony,Bierce's Inheritance"
+ },
+ {
+ "name": "Minanes",
+ "pos_x": 69.875,
+ "pos_y": -143.5625,
+ "pos_z": 36.75,
+ "stations": "Rutan Terminal,Williams Settlement,Eggleton Terminal,Mattei Port"
+ },
+ {
+ "name": "Minang",
+ "pos_x": 157.21875,
+ "pos_y": -48.4375,
+ "pos_z": -43.84375,
+ "stations": "Caillie Settlement"
+ },
+ {
+ "name": "Minarii",
+ "pos_x": 81.0625,
+ "pos_y": 43.9375,
+ "pos_z": 39.125,
+ "stations": "Ferguson Horizons"
+ },
+ {
+ "name": "Minatae",
+ "pos_x": -40.21875,
+ "pos_y": 17.90625,
+ "pos_z": 98.125,
+ "stations": "Shepard Point,Meucci Landing,Cernan Refinery"
+ },
+ {
+ "name": "Minawara",
+ "pos_x": -100.03125,
+ "pos_y": 42.53125,
+ "pos_z": -60.59375,
+ "stations": "Fossum Depot,Margulis Colony,Abnett Prospect"
+ },
+ {
+ "name": "Mindji",
+ "pos_x": -124.4375,
+ "pos_y": -60.0625,
+ "pos_z": -40.71875,
+ "stations": "Goldstein Legacy"
+ },
+ {
+ "name": "Mineer",
+ "pos_x": 54.5625,
+ "pos_y": -21.0625,
+ "pos_z": 60.34375,
+ "stations": "Barth Settlement,Benyovszky Holdings"
+ },
+ {
+ "name": "Minerva",
+ "pos_x": -22.0625,
+ "pos_y": -64.9375,
+ "pos_z": -15.125,
+ "stations": "Starlace Station,Towarnicki,Abasheli Terminal"
+ },
+ {
+ "name": "Mingal",
+ "pos_x": 87.21875,
+ "pos_y": -42.40625,
+ "pos_z": 157.5625,
+ "stations": "Bailey Port,Shunn Orbital,Grothendieck Depot"
+ },
+ {
+ "name": "Mingfu",
+ "pos_x": -8.5,
+ "pos_y": -44.34375,
+ "pos_z": -4.03125,
+ "stations": "Hire Ring"
+ },
+ {
+ "name": "Mingh",
+ "pos_x": -44.375,
+ "pos_y": -33.9375,
+ "pos_z": 152.0625,
+ "stations": "Furukawa Enterprise,Zalyotin Camp"
+ },
+ {
+ "name": "Mingin",
+ "pos_x": -87.9375,
+ "pos_y": 106,
+ "pos_z": 149.09375,
+ "stations": "Finch Port,Reed Platform,Shaw Mines,Moskowitz Hub,Markov Survey"
+ },
+ {
+ "name": "Mingpurine",
+ "pos_x": -130.5,
+ "pos_y": -80.5,
+ "pos_z": 53.78125,
+ "stations": "Filter Orbital,Lee Port,Robson Installation,Swanwick Relay"
+ },
+ {
+ "name": "Minja",
+ "pos_x": 112.875,
+ "pos_y": -143.3125,
+ "pos_z": -7.9375,
+ "stations": "Extra Mines,Youll Enterprise,Hamuy Survey"
+ },
+ {
+ "name": "Minjango",
+ "pos_x": -8.4375,
+ "pos_y": -125.3125,
+ "pos_z": -25.90625,
+ "stations": "Shaver Beacon,Denning Platform,Naboth Vision"
+ },
+ {
+ "name": "Minjanh",
+ "pos_x": 139.46875,
+ "pos_y": -116.15625,
+ "pos_z": 31.40625,
+ "stations": "Wilhelm Klinkerfues Gateway,Carsono Enterprise,McQuarrie Terminal,Kalam Arsenal"
+ },
+ {
+ "name": "Minjul",
+ "pos_x": 86.5,
+ "pos_y": -57.3125,
+ "pos_z": 147.84375,
+ "stations": "Valigursky Landing,Stanley Base"
+ },
+ {
+ "name": "Minjung",
+ "pos_x": -37.5625,
+ "pos_y": -95.65625,
+ "pos_z": -38.96875,
+ "stations": "Shaikh City"
+ },
+ {
+ "name": "Minmar",
+ "pos_x": 14.9375,
+ "pos_y": -20.4375,
+ "pos_z": 8.5625,
+ "stations": "Kandel Hub,Bayliss Landing,Ferguson Landing,Acropolis"
+ },
+ {
+ "name": "Minot",
+ "pos_x": 81.84375,
+ "pos_y": 30.40625,
+ "pos_z": 20.4375,
+ "stations": "Huxley Survey,Williamson City,Cadamosto Terminal,Kurland Hub,Ellison Dock,Ashton Port,Sheckley Port,Abe Dock,Williams Laboratory,Nicolet Terminal"
+ },
+ {
+ "name": "Minu",
+ "pos_x": 37.5625,
+ "pos_y": 139.375,
+ "pos_z": -84.625,
+ "stations": "Cartier Dock,Pierce Dock,Eisenstein Prospect"
+ },
+ {
+ "name": "Minun",
+ "pos_x": -96.5625,
+ "pos_y": 51.4375,
+ "pos_z": 19.6875,
+ "stations": "Shiner Enterprise,Charnas City,Rothman Station,Finch Depot,Grijalva Arsenal,Godwin Prospect"
+ },
+ {
+ "name": "Minung Po",
+ "pos_x": 92.46875,
+ "pos_y": -23.53125,
+ "pos_z": -97.25,
+ "stations": "Poisson Dock,Serling Horizons,Savorgnan de Brazza Dock,Watt-Evans Survey"
+ },
+ {
+ "name": "Miola",
+ "pos_x": -64,
+ "pos_y": 24,
+ "pos_z": -41,
+ "stations": "Harris Hospital,Bernard Market"
+ },
+ {
+ "name": "Miphifa",
+ "pos_x": 60.59375,
+ "pos_y": -114.875,
+ "pos_z": 12.34375,
+ "stations": "Potocnik Vision,Happis,Kludze Lab"
+ },
+ {
+ "name": "Miquich",
+ "pos_x": -17.71875,
+ "pos_y": 19.125,
+ "pos_z": 19.59375,
+ "stations": "Farmer Holdings,Salpeter Enterprise,Onufrienko Hanger"
+ },
+ {
+ "name": "Miquit",
+ "pos_x": 42.9375,
+ "pos_y": -149.875,
+ "pos_z": 48.40625,
+ "stations": "Gonnessiat City,Kraft Vision,Aller City,Wenzel Prospect,Hasse Hub,Leopold Heckmann City,Mason City,Jael Orbital,Rosenberger City,Dingle City,Krigstein Hub,Weill Hub,Meuron Prospect"
+ },
+ {
+ "name": "Miquitagat",
+ "pos_x": 58,
+ "pos_y": -158.625,
+ "pos_z": 1.9375,
+ "stations": "Prandtl Orbital,Gurney Hub,Alfven Ring"
+ },
+ {
+ "name": "Mirateje",
+ "pos_x": -183.15625,
+ "pos_y": 7.75,
+ "pos_z": 41.875,
+ "stations": "Ocampo Station,Heaviside Terminal,Maury Orbital"
+ },
+ {
+ "name": "Miratres",
+ "pos_x": 59.46875,
+ "pos_y": -101.90625,
+ "pos_z": 105.46875,
+ "stations": "Hagihara Station"
+ },
+ {
+ "name": "Mirdhing",
+ "pos_x": 8.15625,
+ "pos_y": 35.71875,
+ "pos_z": -111.71875,
+ "stations": "Cabrera Landing,Bertin Point"
+ },
+ {
+ "name": "Mirdhr",
+ "pos_x": -102.71875,
+ "pos_y": -99.0625,
+ "pos_z": 98.40625,
+ "stations": "Hasse Camp"
+ },
+ {
+ "name": "Mirdi",
+ "pos_x": 41,
+ "pos_y": 1.46875,
+ "pos_z": -66.34375,
+ "stations": "McDivitt Settlement,Kozlov Silo,Sagan Mines"
+ },
+ {
+ "name": "Mirdia",
+ "pos_x": 86.75,
+ "pos_y": -88,
+ "pos_z": 67.1875,
+ "stations": "Mohr Horizons,Yu's Inheritance,Fiorilla Landing"
+ },
+ {
+ "name": "Mirdii",
+ "pos_x": -47.6875,
+ "pos_y": -149.15625,
+ "pos_z": -43,
+ "stations": "Snodgrass' Progress,Gulyaev Platform"
+ },
+ {
+ "name": "Miribusha",
+ "pos_x": 22.8125,
+ "pos_y": 11.125,
+ "pos_z": 40.71875,
+ "stations": "Wright Vision"
+ },
+ {
+ "name": "Miris",
+ "pos_x": -44.5,
+ "pos_y": 89.28125,
+ "pos_z": -62.53125,
+ "stations": "Jeury Beacon,Dukaj Refinery,Heyerdahl Orbital"
+ },
+ {
+ "name": "Miritu",
+ "pos_x": 103.09375,
+ "pos_y": -1.09375,
+ "pos_z": -19.96875,
+ "stations": "Celebi City,Efremov Plant"
+ },
+ {
+ "name": "Miriwung Mu",
+ "pos_x": 91.34375,
+ "pos_y": 77.6875,
+ "pos_z": 6.46875,
+ "stations": "Leavitt Station"
+ },
+ {
+ "name": "Mirnga",
+ "pos_x": 105,
+ "pos_y": -64.3125,
+ "pos_z": -58.75,
+ "stations": "Pinzon's Inheritance,Dupuy de Lome Port"
+ },
+ {
+ "name": "Mirngandin",
+ "pos_x": -22.53125,
+ "pos_y": -186.125,
+ "pos_z": 101.71875,
+ "stations": "Jenkins Port,Haro Vision,Fawcett Vision"
+ },
+ {
+ "name": "Mirolunts",
+ "pos_x": 126.03125,
+ "pos_y": -125.71875,
+ "pos_z": 52.78125,
+ "stations": "Salam Ring"
+ },
+ {
+ "name": "Miroman",
+ "pos_x": 127.5625,
+ "pos_y": -21.125,
+ "pos_z": 61.1875,
+ "stations": "Kazantsev Horizons,Rosse Orbital,Turner Dock,Michalitsianos Hub,Whittle Vision,Zhu Ring,Sargent Palace,Dillon Depot,Steinmuller's Inheritance"
+ },
+ {
+ "name": "Miromi",
+ "pos_x": -3.4375,
+ "pos_y": 59.65625,
+ "pos_z": 115.28125,
+ "stations": "Hamilton Terminal,Saberhagen Gateway,Heyerdahl Port,Wallis Terminal,Piccard Station"
+ },
+ {
+ "name": "Mironen",
+ "pos_x": 77,
+ "pos_y": -207.28125,
+ "pos_z": 111.6875,
+ "stations": "Nicholson Terminal,Shea Terminal,Addams Terminal"
+ },
+ {
+ "name": "Mirongkomi",
+ "pos_x": -58.78125,
+ "pos_y": -1.46875,
+ "pos_z": -93.3125,
+ "stations": "Payette Colony,Morgan Base,Velazquez Holdings,Musabayev Hub,Bailey Horizons"
+ },
+ {
+ "name": "Mironi",
+ "pos_x": 60.3125,
+ "pos_y": -220.4375,
+ "pos_z": 80.9375,
+ "stations": "Coddington Terminal,Langley Port,Priest's Folly"
+ },
+ {
+ "name": "Misao",
+ "pos_x": -60.3125,
+ "pos_y": 0.65625,
+ "pos_z": -122.5,
+ "stations": "Walter's Claim,Quick Installation"
+ },
+ {
+ "name": "Misao Jun",
+ "pos_x": -93.71875,
+ "pos_y": 125.78125,
+ "pos_z": 29.96875,
+ "stations": "Solovyov Colony,Fairbairn Station,Kekule Depot,Bushkov Beacon"
+ },
+ {
+ "name": "Misarret",
+ "pos_x": -105,
+ "pos_y": 67.625,
+ "pos_z": 80.1875,
+ "stations": "Danvers Station,Noether Landing,Gaspar de Lemos Hub,Wilder Installation"
+ },
+ {
+ "name": "Mish",
+ "pos_x": 9.3125,
+ "pos_y": -189.6875,
+ "pos_z": 26.375,
+ "stations": "Matthews Base,Bretnor Barracks"
+ },
+ {
+ "name": "Mishma",
+ "pos_x": 157.09375,
+ "pos_y": 42.5625,
+ "pos_z": 58.8125,
+ "stations": "Aikin's Folly,Marusek Station,Stevens Works"
+ },
+ {
+ "name": "Mishtra",
+ "pos_x": -120.6875,
+ "pos_y": 59.75,
+ "pos_z": 61.65625,
+ "stations": "Landsteiner Terminal,Landsteiner Orbital,Bykovsky City,Perry Prospect"
+ },
+ {
+ "name": "Mising",
+ "pos_x": -144.28125,
+ "pos_y": 74.65625,
+ "pos_z": -20.0625,
+ "stations": "Wingrove Dock,Wright Dock,LeConte's Folly"
+ },
+ {
+ "name": "Misir",
+ "pos_x": 38.125,
+ "pos_y": 12.53125,
+ "pos_z": -48.75,
+ "stations": "Snyder Gateway,Avicenna Enterprise"
+ },
+ {
+ "name": "Misiriang",
+ "pos_x": -144.53125,
+ "pos_y": -47.8125,
+ "pos_z": 97.5,
+ "stations": "Siodmak Refinery,Nehsi Orbital"
+ },
+ {
+ "name": "Misisture",
+ "pos_x": 14.9375,
+ "pos_y": -33.625,
+ "pos_z": 78.09375,
+ "stations": "Gelfand Dock"
+ },
+ {
+ "name": "Misk",
+ "pos_x": 77.4375,
+ "pos_y": -93.5625,
+ "pos_z": -8.1875,
+ "stations": "Paulmier de Gonneville Point"
+ },
+ {
+ "name": "Miskai",
+ "pos_x": -63.3125,
+ "pos_y": -90.875,
+ "pos_z": 59.90625,
+ "stations": "Christopher Installation,Ellis Survey,Noguchi Terminal,Bowersox Park"
+ },
+ {
+ "name": "Mislika",
+ "pos_x": -99.0625,
+ "pos_y": -3.40625,
+ "pos_z": 3.125,
+ "stations": "Saunders Dock"
+ },
+ {
+ "name": "Mistae",
+ "pos_x": -66.28125,
+ "pos_y": 47.71875,
+ "pos_z": -25.875,
+ "stations": "Sargent Dock,Quimper Installation,Mallett Mine"
+ },
+ {
+ "name": "Mistana",
+ "pos_x": -100.375,
+ "pos_y": -0.4375,
+ "pos_z": -58.375,
+ "stations": "Noether Prospect,Bosch Terminal,Korzun Depot"
+ },
+ {
+ "name": "Misthajani",
+ "pos_x": 111.5,
+ "pos_y": -51.21875,
+ "pos_z": 58.15625,
+ "stations": "Encke City,Glushko Dock,Nakano Hub,Freas Works"
+ },
+ {
+ "name": "Misthaka",
+ "pos_x": -56.8125,
+ "pos_y": 85.8125,
+ "pos_z": 65.96875,
+ "stations": "Williams Vision,Hawke Barracks,Penn Installation,Quimper Prospect"
+ },
+ {
+ "name": "Mitaimici",
+ "pos_x": 27.9375,
+ "pos_y": -42.09375,
+ "pos_z": -162.28125,
+ "stations": "Sohl Settlement,Neff's Progress"
+ },
+ {
+ "name": "Mitanja",
+ "pos_x": -10.40625,
+ "pos_y": -174.96875,
+ "pos_z": 23.40625,
+ "stations": "Metcalf Terminal,Courvoisier City"
+ },
+ {
+ "name": "Mitl",
+ "pos_x": -27.5,
+ "pos_y": -16.28125,
+ "pos_z": 96.375,
+ "stations": "Comino Outpost,Bethe Station"
+ },
+ {
+ "name": "Mitlhou Wa",
+ "pos_x": 50.0625,
+ "pos_y": -40.625,
+ "pos_z": 46.6875,
+ "stations": "Eratosthenes Point,Alcala Settlement"
+ },
+ {
+ "name": "Mitnahas",
+ "pos_x": -46.65625,
+ "pos_y": -72.75,
+ "pos_z": -98.4375,
+ "stations": "Burgess Hub,Friend Terminal,Pike Terminal,Bombelli Arsenal,Woolley Station,Tudela Station"
+ },
+ {
+ "name": "Mitra",
+ "pos_x": 37.6875,
+ "pos_y": -39.34375,
+ "pos_z": 5.9375,
+ "stations": "Volterra Horizons,Tokubei Holdings,Resnick Enterprise,Green Observatory,Slusser Station"
+ },
+ {
+ "name": "Mitschigua",
+ "pos_x": 128.4375,
+ "pos_y": -92.96875,
+ "pos_z": 4.34375,
+ "stations": "Pond Installation,McQuarrie Dock,Ashbrook Estate,Crown Works"
+ },
+ {
+ "name": "Miwae",
+ "pos_x": 163.28125,
+ "pos_y": -103.34375,
+ "pos_z": 2.8125,
+ "stations": "Bolkow Platform,Bohr Platform,Watanabe Terminal,Gurevich Depot"
+ },
+ {
+ "name": "Miwanti",
+ "pos_x": 84,
+ "pos_y": 133.875,
+ "pos_z": -35.09375,
+ "stations": "Smith Survey,Doctorow Vision,Lynn Works"
+ },
+ {
+ "name": "Miwobo Kuki",
+ "pos_x": -35.25,
+ "pos_y": -166.71875,
+ "pos_z": 61.90625,
+ "stations": "Herschel Port,Ptack Enterprise,Keyes Base,Kalam Enterprise,Kopff Platform"
+ },
+ {
+ "name": "Miwobogi",
+ "pos_x": 79.03125,
+ "pos_y": -42.9375,
+ "pos_z": 83.03125,
+ "stations": "Donaldson Gateway,Quick Port"
+ },
+ {
+ "name": "Miwodigi",
+ "pos_x": 1.375,
+ "pos_y": 18.03125,
+ "pos_z": -142.8125,
+ "stations": "Rey Station"
+ },
+ {
+ "name": "Mizar",
+ "pos_x": -36.25,
+ "pos_y": 72.8125,
+ "pos_z": -15.46875,
+ "stations": "Webb Camp,Judson Station,Gerlache Arsenal,Bretnor Enterprise"
+ },
+ {
+ "name": "Mizete",
+ "pos_x": -84.25,
+ "pos_y": -96.09375,
+ "pos_z": 67.5,
+ "stations": "Crowley Terminal,Bohnhoff Settlement,Sakers Station,Porges Orbital,Sharp Prospect"
+ },
+ {
+ "name": "Mizo",
+ "pos_x": 35.09375,
+ "pos_y": -130.59375,
+ "pos_z": -30.53125,
+ "stations": "Kerimov Survey,Fuller Colony"
+ },
+ {
+ "name": "Mjolnir",
+ "pos_x": -56.28125,
+ "pos_y": 23.09375,
+ "pos_z": 84.46875,
+ "stations": "Patrick Thomas,Morgan Settlement,Athium"
+ },
+ {
+ "name": "Mo Dadabi",
+ "pos_x": -75.375,
+ "pos_y": 66.53125,
+ "pos_z": -65.6875,
+ "stations": "Low Beacon,Wakata Point"
+ },
+ {
+ "name": "Mo Dadiweu",
+ "pos_x": 90.03125,
+ "pos_y": -106.40625,
+ "pos_z": 154.40625,
+ "stations": "Lozino-Lozinskiy Platform,Schuster Hub,Killough Hub"
+ },
+ {
+ "name": "Mo Daorsemi",
+ "pos_x": 128.875,
+ "pos_y": -47.84375,
+ "pos_z": 85.625,
+ "stations": "Hatanaka Prospect,Auer Bastion,Janifer Beacon,Hirasawa Dock"
+ },
+ {
+ "name": "Mo Disci",
+ "pos_x": 118.75,
+ "pos_y": 96.125,
+ "pos_z": 35.4375,
+ "stations": "du Fresne Platform,Darwin Keep"
+ },
+ {
+ "name": "Moan",
+ "pos_x": 147.84375,
+ "pos_y": 84.96875,
+ "pos_z": -26.0625,
+ "stations": "Young Enterprise,Reynolds Installation,Mitchell Landing,Delany Laboratory,Eudoxus Hub"
+ },
+ {
+ "name": "Moanapiang",
+ "pos_x": 81.125,
+ "pos_y": -22.21875,
+ "pos_z": -67.53125,
+ "stations": "Bradley City,Boole City,Bulychev Base"
+ },
+ {
+ "name": "Moanza",
+ "pos_x": -129.15625,
+ "pos_y": -100.28125,
+ "pos_z": -31.46875,
+ "stations": "Leiber Oasis,McCarthy Prospect,Maclaurin Survey"
+ },
+ {
+ "name": "Moarasi",
+ "pos_x": -59.9375,
+ "pos_y": -96.46875,
+ "pos_z": 63.75,
+ "stations": "Sommerfeld Landing,Lazarev Depot"
+ },
+ {
+ "name": "Moarawai",
+ "pos_x": 119,
+ "pos_y": -74.78125,
+ "pos_z": -30.875,
+ "stations": "Dubyago Plant,Windt Arsenal"
+ },
+ {
+ "name": "Mobia",
+ "pos_x": -9530.6875,
+ "pos_y": -909.84375,
+ "pos_z": 19780.3125,
+ "stations": "Pedersen's Legacy"
+ },
+ {
+ "name": "Mochi",
+ "pos_x": -124.6875,
+ "pos_y": -7.03125,
+ "pos_z": 79.46875,
+ "stations": "Besonders' Inheritance,Fraser Terminal,Siegel Settlement,White Installation"
+ },
+ {
+ "name": "Modgud",
+ "pos_x": 171.875,
+ "pos_y": -111.46875,
+ "pos_z": 2.21875,
+ "stations": "van Rhijn Survey"
+ },
+ {
+ "name": "Modigi",
+ "pos_x": 89.34375,
+ "pos_y": -92.28125,
+ "pos_z": -117.4375,
+ "stations": "Barton Enterprise,Reamy Beacon"
+ },
+ {
+ "name": "Modimo",
+ "pos_x": -114.71875,
+ "pos_y": -22.0625,
+ "pos_z": 124.46875,
+ "stations": "Hui Terminal,Roberts Vista"
+ },
+ {
+ "name": "Moepirt",
+ "pos_x": 30.78125,
+ "pos_y": -14.5625,
+ "pos_z": 25.75,
+ "stations": "Thuot Orbital,Wundt Colony"
+ },
+ {
+ "name": "Moha",
+ "pos_x": -12.375,
+ "pos_y": -81.0625,
+ "pos_z": -68.59375,
+ "stations": "Leonov Mine"
+ },
+ {
+ "name": "Mohan",
+ "pos_x": 167.40625,
+ "pos_y": -141.96875,
+ "pos_z": 97.125,
+ "stations": "Karachkina Oasis,Ptack Hub,Herndon Landing"
+ },
+ {
+ "name": "Mohang O",
+ "pos_x": 128.3125,
+ "pos_y": -84,
+ "pos_z": 84.71875,
+ "stations": "Hall Horizons,Sarmiento de Gamboa Camp"
+ },
+ {
+ "name": "Mohangda",
+ "pos_x": 138.0625,
+ "pos_y": -71.9375,
+ "pos_z": 83.875,
+ "stations": "Vyssotsky Base"
+ },
+ {
+ "name": "Mohara",
+ "pos_x": 104.4375,
+ "pos_y": 22.4375,
+ "pos_z": -111.625,
+ "stations": "Jakes Ring,Platt Ring,Russell Port,Springer Relay,Ryman Relay"
+ },
+ {
+ "name": "Moirai",
+ "pos_x": 34.3125,
+ "pos_y": -44,
+ "pos_z": -15.59375,
+ "stations": "Scoutrix Prime,Rukavishnikov Keep,Russell Relay,Westerfeld Platform"
+ },
+ {
+ "name": "Moko Oh",
+ "pos_x": -17.34375,
+ "pos_y": -4.40625,
+ "pos_z": 134.75,
+ "stations": "Hambly Landing,Shirley Freeport"
+ },
+ {
+ "name": "Mokobidji",
+ "pos_x": -16.625,
+ "pos_y": -10.25,
+ "pos_z": 101.46875,
+ "stations": "Lazarev Mines,Carver Enterprise,Coney Keep"
+ },
+ {
+ "name": "Mokoi",
+ "pos_x": 5.375,
+ "pos_y": -172.40625,
+ "pos_z": -20.09375,
+ "stations": "Boyer Dock,Korlevic Gateway,Orbik Ring,Weinbaum Landing,Maine Silo"
+ },
+ {
+ "name": "Mokojing",
+ "pos_x": 73.4375,
+ "pos_y": 58.34375,
+ "pos_z": -0.21875,
+ "stations": "Noli Terminal,Ferguson Market,Kelleam Gateway,Christian Installation,Raleigh Gateway,Budrys Vista,Benford Relay"
+ },
+ {
+ "name": "Mokosh",
+ "pos_x": -53.3125,
+ "pos_y": 26.28125,
+ "pos_z": 40.90625,
+ "stations": "Lubin Orbital,Euler Survey,Bethe Station,Nicollier Survey"
+ },
+ {
+ "name": "Mokot",
+ "pos_x": -133.90625,
+ "pos_y": 44.90625,
+ "pos_z": 31.96875,
+ "stations": "Bradley Terminal,Eilenberg Station,Samuda Hub,Gunn Survey,al-Khayyam Enterprise,Fernandes Vision"
+ },
+ {
+ "name": "Mokowa",
+ "pos_x": 72.1875,
+ "pos_y": -149.34375,
+ "pos_z": -2.75,
+ "stations": "Volterra Keep,Dhawan Outpost"
+ },
+ {
+ "name": "Mokpanu",
+ "pos_x": -81.375,
+ "pos_y": 37.75,
+ "pos_z": 116.09375,
+ "stations": "Vance Hub,Constantine Prospect"
+ },
+ {
+ "name": "Mokpati",
+ "pos_x": -2.0625,
+ "pos_y": -161,
+ "pos_z": 118.5,
+ "stations": "Rogers Vision,Haro Installation"
+ },
+ {
+ "name": "Moku",
+ "pos_x": 162.0625,
+ "pos_y": -193.15625,
+ "pos_z": 32.125,
+ "stations": "Moore Beacon,Whitney Landing,Rutan Enterprise"
+ },
+ {
+ "name": "Mokujin",
+ "pos_x": 74.71875,
+ "pos_y": -81.8125,
+ "pos_z": -91.5,
+ "stations": "Roskam Platform,Fuller Enterprise"
+ },
+ {
+ "name": "Mokusa",
+ "pos_x": 59.8125,
+ "pos_y": 83.375,
+ "pos_z": 79.15625,
+ "stations": "Erikson Station,McDevitt Station,Stjepan Seljan Station"
+ },
+ {
+ "name": "Mombal",
+ "pos_x": 58.90625,
+ "pos_y": -103.84375,
+ "pos_z": -92.15625,
+ "stations": "Witt Port"
+ },
+ {
+ "name": "Mombaluma",
+ "pos_x": 18.875,
+ "pos_y": -15.84375,
+ "pos_z": 22.03125,
+ "stations": "Goulart Keep,Hughes-Fulford Terminal,Leavitt Orbital,Bennett Colony"
+ },
+ {
+ "name": "Mombari",
+ "pos_x": 52.75,
+ "pos_y": 143.4375,
+ "pos_z": 28.3125,
+ "stations": "Rawn's Claim"
+ },
+ {
+ "name": "Mombawet",
+ "pos_x": 67.25,
+ "pos_y": -42.09375,
+ "pos_z": 20.40625,
+ "stations": "Sterling Ring"
+ },
+ {
+ "name": "Mombilar",
+ "pos_x": 104,
+ "pos_y": 14.5,
+ "pos_z": -54.875,
+ "stations": "Gooch Relay,Hadfield's Claim,Lessing Survey"
+ },
+ {
+ "name": "Mombou",
+ "pos_x": -21.875,
+ "pos_y": 5.75,
+ "pos_z": -158.34375,
+ "stations": "Weitz Survey,Sommerfeld Horizons,Siodmak Base,Wakata Dock"
+ },
+ {
+ "name": "Momoirent",
+ "pos_x": 58.0625,
+ "pos_y": 13.53125,
+ "pos_z": 0.90625,
+ "stations": "Gamow Port,Jahn Dock,Mullane Hub,Kotov Dock"
+ },
+ {
+ "name": "Momotaro",
+ "pos_x": 126.09375,
+ "pos_y": -181.125,
+ "pos_z": 95.34375,
+ "stations": "Cheranovsky Vision"
+ },
+ {
+ "name": "Momovicho",
+ "pos_x": -32,
+ "pos_y": -5.0625,
+ "pos_z": -150.25,
+ "stations": "Ross Port,Drebbel Colony"
+ },
+ {
+ "name": "Mompox",
+ "pos_x": 138.9375,
+ "pos_y": -213.9375,
+ "pos_z": -0.8125,
+ "stations": "Tusi Port,Vaucouleurs Settlement"
+ },
+ {
+ "name": "Momus Reach",
+ "pos_x": -34.9375,
+ "pos_y": -44.15625,
+ "pos_z": -77.34375,
+ "stations": "Tartarus Point,Gagnan Colony,Ralphus,Terry Palace"
+ },
+ {
+ "name": "Mong Kung",
+ "pos_x": -1.34375,
+ "pos_y": 69.4375,
+ "pos_z": 28.125,
+ "stations": "Reightler Hangar,Magnus Landing,Schouten Horizons"
+ },
+ {
+ "name": "Mong O",
+ "pos_x": 9.375,
+ "pos_y": -57.5,
+ "pos_z": -45.96875,
+ "stations": "Potter Holdings"
+ },
+ {
+ "name": "Mong Po",
+ "pos_x": -35.71875,
+ "pos_y": -172.71875,
+ "pos_z": 24.09375,
+ "stations": "Bruck Platform,Battani Terminal,Atwood Beacon"
+ },
+ {
+ "name": "Mongan",
+ "pos_x": -141.09375,
+ "pos_y": -72.625,
+ "pos_z": -1.3125,
+ "stations": "Oswald Dock,Moisuc Station"
+ },
+ {
+ "name": "Mongatha",
+ "pos_x": 34.6875,
+ "pos_y": 37.53125,
+ "pos_z": -47.84375,
+ "stations": "Huygens Plant,Slayton Colony,Mukai Hub"
+ },
+ {
+ "name": "Mongira",
+ "pos_x": -52.84375,
+ "pos_y": -33.5,
+ "pos_z": 146.9375,
+ "stations": "Dorsey Penal colony,Lavrador Settlement"
+ },
+ {
+ "name": "Mongsakah",
+ "pos_x": 145.34375,
+ "pos_y": -35.3125,
+ "pos_z": 11.875,
+ "stations": "Baynes Camp"
+ },
+ {
+ "name": "Monjadi",
+ "pos_x": 57.65625,
+ "pos_y": 3.625,
+ "pos_z": 139.71875,
+ "stations": "Noriega Port,Solovyov Plant,Carey Landing,Rochon Installation,Leckie Enterprise"
+ },
+ {
+ "name": "Monjiwatka",
+ "pos_x": 96.375,
+ "pos_y": -237.1875,
+ "pos_z": 40.84375,
+ "stations": "Puleston Survey,Hawker Mine,Vyssotsky Terminal"
+ },
+ {
+ "name": "Mono",
+ "pos_x": -115.625,
+ "pos_y": -112.59375,
+ "pos_z": -57.03125,
+ "stations": "Le Guin Plant"
+ },
+ {
+ "name": "Monosus",
+ "pos_x": 138.0625,
+ "pos_y": -22.96875,
+ "pos_z": -2.53125,
+ "stations": "Evans Vista,Al-Farabi Landing"
+ },
+ {
+ "name": "Monoto",
+ "pos_x": 57.59375,
+ "pos_y": -33.1875,
+ "pos_z": 33.90625,
+ "stations": "Reed Dock,Zoline Dock,Chebyshev Installation,Altshuller Port,Gubarev's Progress"
+ },
+ {
+ "name": "Mons",
+ "pos_x": 118.8125,
+ "pos_y": -17.21875,
+ "pos_z": 125.9375,
+ "stations": "Vardeman Vision,Bushkov Horizons,Macedo Hub"
+ },
+ {
+ "name": "Monsu",
+ "pos_x": 66.75,
+ "pos_y": 13.84375,
+ "pos_z": 133.53125,
+ "stations": "Daimler Survey,Werner von Siemens Settlement"
+ },
+ {
+ "name": "Mont",
+ "pos_x": -90.21875,
+ "pos_y": -83.71875,
+ "pos_z": 138.9375,
+ "stations": "Hugh Holdings,Bethke Lab"
+ },
+ {
+ "name": "Montet",
+ "pos_x": -7.59375,
+ "pos_y": 1.4375,
+ "pos_z": 165.09375,
+ "stations": "Seddon Gateway,Thirsk Enterprise"
+ },
+ {
+ "name": "Montidi",
+ "pos_x": 85.1875,
+ "pos_y": 21.625,
+ "pos_z": 141.65625,
+ "stations": "Krylov Landing,Ziljak Landing"
+ },
+ {
+ "name": "Montino",
+ "pos_x": -120.75,
+ "pos_y": 56.84375,
+ "pos_z": 56,
+ "stations": "Kovalevskaya Colony,Pohl Legacy"
+ },
+ {
+ "name": "Montioch",
+ "pos_x": 166.9375,
+ "pos_y": -85.875,
+ "pos_z": -43.40625,
+ "stations": "Arp Arena"
+ },
+ {
+ "name": "Monto",
+ "pos_x": -67.65625,
+ "pos_y": -54.03125,
+ "pos_z": 16.84375,
+ "stations": "Werber Hangar,Adams' Folly,Pytheas Landing"
+ },
+ {
+ "name": "Montovici",
+ "pos_x": 92.40625,
+ "pos_y": 57.5625,
+ "pos_z": -41.15625,
+ "stations": "Quick Hub,Abbott City,Taylor Hub,Butler Orbital,Vonarburg Gateway,Atwood's Inheritance,Selous Works,Malaspina City,Werber Terminal,King Hub,Noether Settlement"
+ },
+ {
+ "name": "Mool",
+ "pos_x": 92.40625,
+ "pos_y": -179.0625,
+ "pos_z": -16.4375,
+ "stations": "Gasparis Terminal,Paola Dock,Pearse Refinery,Fan Terminal,Kuttner Prospect"
+ },
+ {
+ "name": "Moora",
+ "pos_x": 57.625,
+ "pos_y": 18.1875,
+ "pos_z": -145.9375,
+ "stations": "Tasman City,Bryant Station,Chaviano Enterprise,Yeliseyev's Inheritance,Preuss Holdings"
+ },
+ {
+ "name": "Mooramba",
+ "pos_x": -68.625,
+ "pos_y": -17.5,
+ "pos_z": -149.125,
+ "stations": "Timofeyevich Point"
+ },
+ {
+ "name": "Mooramoora",
+ "pos_x": 41.15625,
+ "pos_y": 124.03125,
+ "pos_z": -71.03125,
+ "stations": "Rzeppa Gateway"
+ },
+ {
+ "name": "Moortic",
+ "pos_x": -73.21875,
+ "pos_y": 49.96875,
+ "pos_z": 12.78125,
+ "stations": "DeLucas Hangar,Stott Station,Bluford Settlement,Descartes Settlement"
+ },
+ {
+ "name": "Mopadigi",
+ "pos_x": 61.84375,
+ "pos_y": -87.9375,
+ "pos_z": 146.28125,
+ "stations": "Penrose Orbital,Wolszczan Dock,Karachkina Platform,Vasilyev Refinery,Hinz Base"
+ },
+ {
+ "name": "Mopadil",
+ "pos_x": -90.96875,
+ "pos_y": -92.1875,
+ "pos_z": 48.71875,
+ "stations": "Bunch Terminal,Forward Dock"
+ },
+ {
+ "name": "Mopaditis",
+ "pos_x": 63.0625,
+ "pos_y": -67.78125,
+ "pos_z": 102.875,
+ "stations": "Thiele City,Lilienthal Dock,Mayor Port,Roberts Lab"
+ },
+ {
+ "name": "Mopanekpen",
+ "pos_x": -78.75,
+ "pos_y": -41.46875,
+ "pos_z": -115.625,
+ "stations": "Salam Beacon"
+ },
+ {
+ "name": "Mopanyane",
+ "pos_x": -22.96875,
+ "pos_y": -11.59375,
+ "pos_z": 80.65625,
+ "stations": "Bischoff Station,Pangborn Dock,Bulgarin Dock"
+ },
+ {
+ "name": "Mora",
+ "pos_x": -154.03125,
+ "pos_y": -54.875,
+ "pos_z": -20.875,
+ "stations": "Lazutkin Port,Worden Hub,Jemison Installation,George Beacon"
+ },
+ {
+ "name": "Morai",
+ "pos_x": 49.53125,
+ "pos_y": -92.28125,
+ "pos_z": -10.59375,
+ "stations": "van de Hulst Vision"
+ },
+ {
+ "name": "Moraikda",
+ "pos_x": -142.59375,
+ "pos_y": -77.875,
+ "pos_z": -109.90625,
+ "stations": "North Outpost"
+ },
+ {
+ "name": "Moraitha",
+ "pos_x": -102.53125,
+ "pos_y": 99.90625,
+ "pos_z": 37.125,
+ "stations": "Williams Prospect,Boole Terminal"
+ },
+ {
+ "name": "Moraja",
+ "pos_x": 22.125,
+ "pos_y": -44.40625,
+ "pos_z": 115.5625,
+ "stations": "Bradley Dock,Heinlein Outpost"
+ },
+ {
+ "name": "Moram",
+ "pos_x": 143.1875,
+ "pos_y": -13.65625,
+ "pos_z": 0.375,
+ "stations": "Ziemianski Dock,Tapinas Ring"
+ },
+ {
+ "name": "Morana",
+ "pos_x": 92.53125,
+ "pos_y": -95.59375,
+ "pos_z": 11.34375,
+ "stations": "G-Mahoney-1,Farrer Colony,Seares Station,Lenthall's Inheritance,Brouwer Depot,Kojima's Inheritance"
+ },
+ {
+ "name": "Morgor",
+ "pos_x": -15.25,
+ "pos_y": 39.53125,
+ "pos_z": -2.25,
+ "stations": "Romanek's Folly,Sekelj Forum"
+ },
+ {
+ "name": "Moria Bahpu",
+ "pos_x": 144.96875,
+ "pos_y": -1.75,
+ "pos_z": -10.375,
+ "stations": "Heck Sanctuary"
+ },
+ {
+ "name": "Morinbath",
+ "pos_x": 43.59375,
+ "pos_y": 42.34375,
+ "pos_z": -74.375,
+ "stations": "Drzewiecki Terminal,Klink Base,Gordon Refinery,McCormick Relay"
+ },
+ {
+ "name": "Morinja",
+ "pos_x": 59.0625,
+ "pos_y": -38.5,
+ "pos_z": 145.40625,
+ "stations": "Janjetov Camp,Fairey's Inheritance"
+ },
+ {
+ "name": "Morinmutino",
+ "pos_x": 135.875,
+ "pos_y": -155.90625,
+ "pos_z": 52.03125,
+ "stations": "Parker Mine"
+ },
+ {
+ "name": "Moriongo",
+ "pos_x": -102.28125,
+ "pos_y": 7.78125,
+ "pos_z": -114.6875,
+ "stations": "Baker Survey"
+ },
+ {
+ "name": "Morios",
+ "pos_x": -3.28125,
+ "pos_y": 144.65625,
+ "pos_z": -96.34375,
+ "stations": "Asaro Plant,Siegel Port,Macedo's Folly"
+ },
+ {
+ "name": "Moriosong",
+ "pos_x": 92.96875,
+ "pos_y": -53.9375,
+ "pos_z": -60.34375,
+ "stations": "Ford City,Kwolek City,Brady Prospect,Fife Installation,Sucharitkul Depot"
+ },
+ {
+ "name": "Morioux",
+ "pos_x": -41.78125,
+ "pos_y": -137.0625,
+ "pos_z": 113.875,
+ "stations": "Bond Port"
+ },
+ {
+ "name": "Morixa",
+ "pos_x": 56.03125,
+ "pos_y": 19.71875,
+ "pos_z": -70.09375,
+ "stations": "Wetherbee Gateway,Tokarev City,Makarov Port,Fontana Ring,Smith Port,Wakata Port,Bresnik Port,Bear Enterprise,Anderson Beacon,Nesvadba Installation,Alexander Terminal,Leichhardt Installation,Morrison Holdings,Montrose Survey"
+ },
+ {
+ "name": "Moroecani",
+ "pos_x": 40.09375,
+ "pos_y": 52.59375,
+ "pos_z": -17.21875,
+ "stations": "Hopkins Retreat"
+ },
+ {
+ "name": "Morommo",
+ "pos_x": 97.125,
+ "pos_y": 10.90625,
+ "pos_z": -25.96875,
+ "stations": "Aubakirov Laboratory,Reed Station,Artin Hub"
+ },
+ {
+ "name": "Morong",
+ "pos_x": -14.46875,
+ "pos_y": 39,
+ "pos_z": -169.625,
+ "stations": "Parry Penal colony,Stephenson Vision,Rowley Hub"
+ },
+ {
+ "name": "Moroni",
+ "pos_x": 128.59375,
+ "pos_y": -37.46875,
+ "pos_z": -7.59375,
+ "stations": "Glenn Vision"
+ },
+ {
+ "name": "Moronit",
+ "pos_x": -83.78125,
+ "pos_y": -120.46875,
+ "pos_z": -19.75,
+ "stations": "Hamilton Plant,Crumey Camp"
+ },
+ {
+ "name": "Moror",
+ "pos_x": -112.28125,
+ "pos_y": 12.15625,
+ "pos_z": 92.03125,
+ "stations": "Borel Installation,Drebbel Port"
+ },
+ {
+ "name": "Moros",
+ "pos_x": -45.96875,
+ "pos_y": 17.375,
+ "pos_z": -31.3125,
+ "stations": "Dana Port,Watt Terminal,Ellis Port"
+ },
+ {
+ "name": "Morotep",
+ "pos_x": 131.90625,
+ "pos_y": -176.1875,
+ "pos_z": -20.84375,
+ "stations": "Michell Terminal,Babcock Colony,Barcelona Point"
+ },
+ {
+ "name": "Morotriman",
+ "pos_x": 92.90625,
+ "pos_y": 59.375,
+ "pos_z": 58.46875,
+ "stations": "Einstein Dock,Baydukov Barracks"
+ },
+ {
+ "name": "Morotrimpe",
+ "pos_x": -33.5625,
+ "pos_y": 88.53125,
+ "pos_z": 78.96875,
+ "stations": "Napier Ring,Payton Port,Gooch Dock,Fabian Gateway,Yurchikhin City,Favier Port,Chilton City"
+ },
+ {
+ "name": "Morowari",
+ "pos_x": -47.5625,
+ "pos_y": -75,
+ "pos_z": -173.1875,
+ "stations": "Moorcock Depot,Bulychev Stop,Springer Keep"
+ },
+ {
+ "name": "Morpheus",
+ "pos_x": -9543.96875,
+ "pos_y": -892.9375,
+ "pos_z": 19808.34375,
+ "stations": "West Base"
+ },
+ {
+ "name": "Morra",
+ "pos_x": 86.03125,
+ "pos_y": -101.4375,
+ "pos_z": 102.25,
+ "stations": "Lassell Dock,Lindblad Enterprise,MacCurdy Base,MacLeod Barracks"
+ },
+ {
+ "name": "Morri",
+ "pos_x": 0.65625,
+ "pos_y": 104.59375,
+ "pos_z": -137.125,
+ "stations": "Tsibliyev Mine,Dobrovolskiy Beacon"
+ },
+ {
+ "name": "Morrigan",
+ "pos_x": -80.40625,
+ "pos_y": 59.53125,
+ "pos_z": -91.53125,
+ "stations": "Tryggvason City,Reilly Port,Cugnot Orbital,Pashin Landing"
+ },
+ {
+ "name": "Morrina",
+ "pos_x": -97.65625,
+ "pos_y": 81.96875,
+ "pos_z": 0.4375,
+ "stations": "Penn Orbital,Barjavel Vision,Beaumont Dock,Moore Base,Mukai's Pride,J. G. Ballard Terminal,Holberg Station,Davidson Hub,Landis' Inheritance"
+ },
+ {
+ "name": "Morronii",
+ "pos_x": -56.53125,
+ "pos_y": -79.59375,
+ "pos_z": 71.09375,
+ "stations": "Linenger Landing,Wheelock Terminal,Gubarev Port,Bryusov Reach,Jacquard Colony"
+ },
+ {
+ "name": "Mors",
+ "pos_x": 99.40625,
+ "pos_y": -57,
+ "pos_z": -21.625,
+ "stations": "Abetti Vision,Foerster City,Cartier Prospect"
+ },
+ {
+ "name": "Morta",
+ "pos_x": -48.3125,
+ "pos_y": -27.875,
+ "pos_z": 17.09375,
+ "stations": "Franke Freeport"
+ },
+ {
+ "name": "Mortel",
+ "pos_x": -22.46875,
+ "pos_y": 73.78125,
+ "pos_z": 44.09375,
+ "stations": "Murdoch Orbital,Kimbrough Dock,Dashiell Relay,Glashow Orbital,Coulter Legacy,Wilmore Dock,Readdy Holdings,Lasswitz Park,Velazquez Station"
+ },
+ {
+ "name": "Morten-Marte",
+ "pos_x": 81.875,
+ "pos_y": 36.875,
+ "pos_z": 47.53125,
+ "stations": "James Sneddon"
+ },
+ {
+ "name": "Moscabs",
+ "pos_x": -3.875,
+ "pos_y": -141.71875,
+ "pos_z": -84.875,
+ "stations": "Grant Survey,Einstein Dock,White Works"
+ },
+ {
+ "name": "Mosche",
+ "pos_x": 69.9375,
+ "pos_y": -36.1875,
+ "pos_z": 92.90625,
+ "stations": "Cortes Hub,Gaiman Orbital,Harness Keep,Auld Dock,Bayliss Beacon"
+ },
+ {
+ "name": "Moshi",
+ "pos_x": 98.53125,
+ "pos_y": -92.78125,
+ "pos_z": -82.5625,
+ "stations": "Shavyrin Refinery,Christy Station"
+ },
+ {
+ "name": "Mosho",
+ "pos_x": 72.65625,
+ "pos_y": -142.375,
+ "pos_z": 122.84375,
+ "stations": "Hoerner Outpost"
+ },
+ {
+ "name": "Mossarian",
+ "pos_x": -11.21875,
+ "pos_y": -110.90625,
+ "pos_z": 127.71875,
+ "stations": "Lemaitre Terminal,Fabricius Oasis"
+ },
+ {
+ "name": "Motia",
+ "pos_x": 112.125,
+ "pos_y": -59.90625,
+ "pos_z": 53.84375,
+ "stations": "Cartier Dock,Pellegrino Point,O'Neill Port"
+ },
+ {
+ "name": "Motie",
+ "pos_x": 96,
+ "pos_y": 50.125,
+ "pos_z": 26.40625,
+ "stations": "Lerner Terminal,Bishop Installation"
+ },
+ {
+ "name": "Motilekui",
+ "pos_x": 115.9375,
+ "pos_y": -244.65625,
+ "pos_z": -64.875,
+ "stations": "Schwabe Terminal,Young Ring,Curtis Park,Abell Keep"
+ },
+ {
+ "name": "Motilone",
+ "pos_x": 131.0625,
+ "pos_y": -59.5625,
+ "pos_z": 76.375,
+ "stations": "Struve Station,Hay Oasis,Dantec Enterprise"
+ },
+ {
+ "name": "Motintana",
+ "pos_x": 125.0625,
+ "pos_y": -166.65625,
+ "pos_z": -59.5625,
+ "stations": "McKie Dock"
+ },
+ {
+ "name": "Moyopa",
+ "pos_x": 70,
+ "pos_y": -199.875,
+ "pos_z": -8.34375,
+ "stations": "Axon Survey,Vuia Mines,Serrao Beacon"
+ },
+ {
+ "name": "Moyot",
+ "pos_x": 24.8125,
+ "pos_y": -260.65625,
+ "pos_z": 12.78125,
+ "stations": "Bowell City,de Seversky Port,Harrington Orbital,Arnason's Progress"
+ },
+ {
+ "name": "Mpalans",
+ "pos_x": 122.875,
+ "pos_y": -10,
+ "pos_z": -98.1875,
+ "stations": "Barentsz Freeport,Napier Base,Morris Arena"
+ },
+ {
+ "name": "Mpalital",
+ "pos_x": 48.21875,
+ "pos_y": -67.8125,
+ "pos_z": -96.15625,
+ "stations": "Metz Dock"
+ },
+ {
+ "name": "Mpalouros",
+ "pos_x": 91.21875,
+ "pos_y": -61.75,
+ "pos_z": -76.5,
+ "stations": "Scott Point,Buffett Survey"
+ },
+ {
+ "name": "Mriya",
+ "pos_x": -9515.4375,
+ "pos_y": -905.75,
+ "pos_z": 19830.8125,
+ "stations": "Taras Shevchenko Hub"
+ },
+ {
+ "name": "MS Draconis",
+ "pos_x": -72.71875,
+ "pos_y": 45.40625,
+ "pos_z": -5.875,
+ "stations": "Piper Holdings,Lunan Beacon"
+ },
+ {
+ "name": "Mse",
+ "pos_x": -99.125,
+ "pos_y": 86.3125,
+ "pos_z": 88.6875,
+ "stations": "Mourelle Landing,Taylor Silo"
+ },
+ {
+ "name": "MT Pegasi",
+ "pos_x": -65.75,
+ "pos_y": -46.625,
+ "pos_z": -2.65625,
+ "stations": "Gurney Holdings,Hubble Base,Ferguson Port"
+ },
+ {
+ "name": "Mu Andromedae",
+ "pos_x": -97.4375,
+ "pos_y": -52.9375,
+ "pos_z": -67.375,
+ "stations": "Lee Outpost,Ziemianski Point"
+ },
+ {
+ "name": "Mu Aquarii",
+ "pos_x": -85.125,
+ "pos_y": -82.15625,
+ "pos_z": 103.625,
+ "stations": "Ivanishin Dock,Fung Hub,LeConte's Inheritance"
+ },
+ {
+ "name": "Mu Aquilae",
+ "pos_x": -75.34375,
+ "pos_y": -12.1875,
+ "pos_z": 75.84375,
+ "stations": "Curie Hub,Ray Horizons,Hale Dock"
+ },
+ {
+ "name": "Mu Arae",
+ "pos_x": 16.625,
+ "pos_y": -10.5625,
+ "pos_z": 46.59375,
+ "stations": "Herreshoff Hangar"
+ },
+ {
+ "name": "Mu Capricorni",
+ "pos_x": -40.21875,
+ "pos_y": -63.34375,
+ "pos_z": 43.6875,
+ "stations": "Somerset Settlement,Chang-Diaz Colony"
+ },
+ {
+ "name": "Mu Gong",
+ "pos_x": 126.1875,
+ "pos_y": -141.4375,
+ "pos_z": 107.40625,
+ "stations": "Kraft Orbital,Nomura Orbital,Siegel Station,Bliss Port,Marius Vision,Safdie Vision,Cyrrhus Vision,Naubakht Vision,Pond Forum"
+ },
+ {
+ "name": "Mu Horologii",
+ "pos_x": 89.625,
+ "pos_y": -109,
+ "pos_z": 11.0625,
+ "stations": "Seares Port,Leberecht Tempel Station"
+ },
+ {
+ "name": "Mu Kin",
+ "pos_x": -171.46875,
+ "pos_y": -74.84375,
+ "pos_z": -62.78125,
+ "stations": "Brust Relay"
+ },
+ {
+ "name": "Mu Koji",
+ "pos_x": 124.65625,
+ "pos_y": -24.71875,
+ "pos_z": 62.46875,
+ "stations": "Thuot Market,Phillips Dock,Aksyonov Hub,Tesla Station,Burnham Lab,McCoy Hub,Voss City,Carr Dock,Thornycroft Enterprise"
+ },
+ {
+ "name": "Mu Nialfi",
+ "pos_x": 32.8125,
+ "pos_y": -215.21875,
+ "pos_z": -43.34375,
+ "stations": "Freas Stop,DiFate Landing,McCrea Works"
+ },
+ {
+ "name": "Mu Nik",
+ "pos_x": -11.28125,
+ "pos_y": -86.96875,
+ "pos_z": 102.96875,
+ "stations": "McArthur Works"
+ },
+ {
+ "name": "Mu Nora",
+ "pos_x": -115.09375,
+ "pos_y": 43.84375,
+ "pos_z": -24.5,
+ "stations": "Mondeh Base,Savitskaya's Claim"
+ },
+ {
+ "name": "Mu Phoenicis",
+ "pos_x": 62.9375,
+ "pos_y": -232.78125,
+ "pos_z": 47.6875,
+ "stations": "Milne Port,Kanai Port,Addams Vision"
+ },
+ {
+ "name": "Mu Telescopii",
+ "pos_x": 29.625,
+ "pos_y": -52.625,
+ "pos_z": 94.46875,
+ "stations": "Weil Orbital,Qian Bastion"
+ },
+ {
+ "name": "Mu Velorum",
+ "pos_x": 112.65625,
+ "pos_y": 17.3125,
+ "pos_z": 26.96875,
+ "stations": "Cook Dock,Akers Hub,Bierce Plant,de Sousa Base"
+ },
+ {
+ "name": "Mu-1 Cygni",
+ "pos_x": -67.96875,
+ "pos_y": -23.03125,
+ "pos_z": 10.59375,
+ "stations": "Furrer Gateway"
+ },
+ {
+ "name": "Mu-1 Pavonis",
+ "pos_x": 104.125,
+ "pos_y": -126.65625,
+ "pos_z": 173.8125,
+ "stations": "Theodorsen Colony,Lambert Vision"
+ },
+ {
+ "name": "Mu-2 Octantis",
+ "pos_x": 78.5625,
+ "pos_y": -78.375,
+ "pos_z": 88.78125,
+ "stations": "Soukup Silo,Chiang Orbital,Hyakutake City,Coblentz Survey"
+ },
+ {
+ "name": "Muan",
+ "pos_x": 89.03125,
+ "pos_y": -236.8125,
+ "pos_z": -44.90625,
+ "stations": "Dufay Station,Adelman Mines,Salgari Enterprise"
+ },
+ {
+ "name": "Muan Nu",
+ "pos_x": 99,
+ "pos_y": -63.5,
+ "pos_z": -49.96875,
+ "stations": "Barnes Relay,Silves Port,Dalmas Station"
+ },
+ {
+ "name": "Muan Qingga",
+ "pos_x": -28.53125,
+ "pos_y": 9.25,
+ "pos_z": 172.15625,
+ "stations": "Makeev Vision,Lethem Port,Merchiston Holdings"
+ },
+ {
+ "name": "Muang",
+ "pos_x": 17.03125,
+ "pos_y": -172.78125,
+ "pos_z": -3.46875,
+ "stations": "Broo's Legacy,Al-Khujandi Enterprise,Hyakutake Settlement,Morgan Terminal,Karlsefni Prospect,Stasheff Prospect"
+ },
+ {
+ "name": "Much",
+ "pos_x": 156.21875,
+ "pos_y": -158.875,
+ "pos_z": -23.71875,
+ "stations": "Palisa Orbital,Efremov's Inheritance,Filippenko Hub,Everest Mine"
+ },
+ {
+ "name": "Muchihiks",
+ "pos_x": -80,
+ "pos_y": 36.9375,
+ "pos_z": -80.53125,
+ "stations": "Gaffney Ring,Levi-Montalcini Terminal,Stephenson Arena,Nagel Relay"
+ },
+ {
+ "name": "Mudhrid",
+ "pos_x": 42.8125,
+ "pos_y": -89.75,
+ "pos_z": -41.25,
+ "stations": "Wachmann Installation,Payne-Scott City,Daqing Terminal"
+ },
+ {
+ "name": "Mudrama Kaze",
+ "pos_x": 97.9375,
+ "pos_y": -110.5625,
+ "pos_z": -54.1875,
+ "stations": "Piazzi Vision,Israel Port,Brin Beacon,Crown Plant,Iwamoto Station"
+ },
+ {
+ "name": "Mudrus",
+ "pos_x": -43.65625,
+ "pos_y": -109.90625,
+ "pos_z": 16.34375,
+ "stations": "Niemeyer Station,Bonestell Platform,Brunner Reach"
+ },
+ {
+ "name": "Muduma",
+ "pos_x": -16.03125,
+ "pos_y": 121.40625,
+ "pos_z": 53.28125,
+ "stations": "Dalmas Mines"
+ },
+ {
+ "name": "Mudumboja",
+ "pos_x": -48.15625,
+ "pos_y": 102.71875,
+ "pos_z": -75.125,
+ "stations": "Simmons Refinery,Conklin Refinery"
+ },
+ {
+ "name": "Mudungkala",
+ "pos_x": 62.4375,
+ "pos_y": 102.4375,
+ "pos_z": -37.96875,
+ "stations": "Jendrassik Port,Skvortsov Enterprise"
+ },
+ {
+ "name": "Mudusitoq",
+ "pos_x": -47.71875,
+ "pos_y": -84.0625,
+ "pos_z": -90.53125,
+ "stations": "Andrews Base,Daimler Gateway,Hadfield Orbital,Haldeman II Arsenal"
+ },
+ {
+ "name": "Muduwa",
+ "pos_x": 164.40625,
+ "pos_y": -129.59375,
+ "pos_z": 133.9375,
+ "stations": "Dall Depot,Schwabe Station,Opik Depot"
+ },
+ {
+ "name": "Mufrid",
+ "pos_x": -1.125,
+ "pos_y": 35.25,
+ "pos_z": 11.09375,
+ "stations": "Shepherd Vision,Boas Orbital,Cheli Station"
+ },
+ {
+ "name": "Muga",
+ "pos_x": 71.40625,
+ "pos_y": -216.40625,
+ "pos_z": 29.5,
+ "stations": "Whelan Relay"
+ },
+ {
+ "name": "Mugari",
+ "pos_x": 110.5,
+ "pos_y": -61.40625,
+ "pos_z": 117.25,
+ "stations": "Fuller Terminal,Fukushima Oasis"
+ },
+ {
+ "name": "Mukh",
+ "pos_x": 164.21875,
+ "pos_y": -156.5,
+ "pos_z": 46,
+ "stations": "Secchi Survey,Kawasato Terminal,Herschel Reach"
+ },
+ {
+ "name": "Mukhang",
+ "pos_x": 162.46875,
+ "pos_y": -200.25,
+ "pos_z": 44.96875,
+ "stations": "Bass' Inheritance,Neugebauer Hub,Nouvel Terminal,Verrier City,Sudworth Vision"
+ },
+ {
+ "name": "Muku",
+ "pos_x": 116.9375,
+ "pos_y": -18.09375,
+ "pos_z": 68.9375,
+ "stations": "Nordenskiold Refinery,Gilliland Vision"
+ },
+ {
+ "name": "Mukulcana",
+ "pos_x": 19.28125,
+ "pos_y": 28.3125,
+ "pos_z": -137.15625,
+ "stations": "Ross Outpost,Gloss Dock"
+ },
+ {
+ "name": "Mukun",
+ "pos_x": -40.9375,
+ "pos_y": 31.15625,
+ "pos_z": -100.25,
+ "stations": "Gaspar de Lemos Laboratory,Weiss Penal colony,Varthema Reformatory"
+ },
+ {
+ "name": "Mukur",
+ "pos_x": 154.21875,
+ "pos_y": -52.96875,
+ "pos_z": 37.6875,
+ "stations": "Calatrava Orbital,Tsiolkovsky Vision"
+ },
+ {
+ "name": "Mukurumnon",
+ "pos_x": 101.90625,
+ "pos_y": -124.8125,
+ "pos_z": -61.34375,
+ "stations": "Hartmann Port,Hague Platform,Gurshtein Station"
+ },
+ {
+ "name": "Mukusubii",
+ "pos_x": -147.46875,
+ "pos_y": -64.125,
+ "pos_z": 46.09375,
+ "stations": "Ledyard Dock,Lasswitz Silo,Ostrander Platform,Dantec Platform"
+ },
+ {
+ "name": "Mula Wendes",
+ "pos_x": 15.71875,
+ "pos_y": -164.5,
+ "pos_z": 86.34375,
+ "stations": "Helin Station,Orbik Hub,Arnold Schwassmann Station,Tange Orbital,Shoemaker Station,Effinger Prospect,Vyssotsky Terminal,Osterbrock Hub,Giunta Orbital,Fan Colony,Bohr Forum,Kurland's Progress,Millosevich Dock,Jackson Keep,Roche's Progress"
+ },
+ {
+ "name": "Mulac",
+ "pos_x": 0.90625,
+ "pos_y": 99.1875,
+ "pos_z": 100.90625,
+ "stations": "Benz City,Coke Enterprise,Wrangell Port"
+ },
+ {
+ "name": "Mulachi",
+ "pos_x": 6.25,
+ "pos_y": -5.78125,
+ "pos_z": 35.9375,
+ "stations": "Shepard Enterprise,Akers Market,Clark Terminal,Gernhardt Terminal,Jemison's Pride,Cauchy Installation,Fleming City,Dalton Orbital,Clark Hub,Laue City,Simonyi Enterprise,Eisenstein Plant"
+ },
+ {
+ "name": "Mulano",
+ "pos_x": -139.96875,
+ "pos_y": 67.5,
+ "pos_z": -15.5625,
+ "stations": "Cousteau's Claim,Tarentum Dock,Shaver Base"
+ },
+ {
+ "name": "Mulaya",
+ "pos_x": 133.09375,
+ "pos_y": -31.59375,
+ "pos_z": 88,
+ "stations": "Gelfand Base,Ulloa Base"
+ },
+ {
+ "name": "Mulayo",
+ "pos_x": 33.875,
+ "pos_y": 9.28125,
+ "pos_z": 97.375,
+ "stations": "Veach Terminal,McKay Gateway,Favier Station,Arnold Terminal"
+ },
+ {
+ "name": "Mullag",
+ "pos_x": 0.5,
+ "pos_y": 35.09375,
+ "pos_z": -19.1875,
+ "stations": "Potagos Station,Hume Orbital,Popov Ring,Kippax Gateway,Bulychev Ring,Katzenstein Landing,Hawkes Horizons,Garfinkle City,Vercors Station,Clough Prospect,McAuley Orbital,Reamy Installation,Akers Keep,al-Haytham Vision,Quick Orbital"
+ },
+ {
+ "name": "Multure",
+ "pos_x": 32.78125,
+ "pos_y": -155.875,
+ "pos_z": 63,
+ "stations": "Prandtl Point,Bell Prospect"
+ },
+ {
+ "name": "Multuring",
+ "pos_x": -59.125,
+ "pos_y": -164.09375,
+ "pos_z": 3.6875,
+ "stations": "Brorsen Port,Porco Ring,Nomen Port,Jones Installation,Shunn Mine"
+ },
+ {
+ "name": "Mulukang",
+ "pos_x": 70.3125,
+ "pos_y": 68.40625,
+ "pos_z": 71.6875,
+ "stations": "al-Khowarizmi Relay"
+ },
+ {
+ "name": "Mulung",
+ "pos_x": -75.875,
+ "pos_y": 86.375,
+ "pos_z": 26.4375,
+ "stations": "Hill Station,Okorafor Camp,Navigator Penal colony"
+ },
+ {
+ "name": "Mulung Piri",
+ "pos_x": -30.5625,
+ "pos_y": 157.8125,
+ "pos_z": -2.21875,
+ "stations": "Peirce Mine,Grego Relay"
+ },
+ {
+ "name": "Mulungu",
+ "pos_x": -36.5,
+ "pos_y": -52.125,
+ "pos_z": 12.15625,
+ "stations": "Feynman Port,Pawelczyk Orbital"
+ },
+ {
+ "name": "Mumba",
+ "pos_x": 82.34375,
+ "pos_y": 170.5,
+ "pos_z": -94.875,
+ "stations": "Priest Base"
+ },
+ {
+ "name": "Mumbal",
+ "pos_x": 29.65625,
+ "pos_y": -61.0625,
+ "pos_z": 86.9375,
+ "stations": "Penn Penal colony,Jones Dock,Adragna Station"
+ },
+ {
+ "name": "Mumbiku",
+ "pos_x": -22.03125,
+ "pos_y": -141.96875,
+ "pos_z": -61.78125,
+ "stations": "Rees Mine,Bradley Depot"
+ },
+ {
+ "name": "Mumukulas",
+ "pos_x": -3.3125,
+ "pos_y": -120.40625,
+ "pos_z": -97.78125,
+ "stations": "Pogue Platform,Rozhdestvensky Mine"
+ },
+ {
+ "name": "Muncheim",
+ "pos_x": -98.375,
+ "pos_y": 37.5625,
+ "pos_z": -61.28125,
+ "stations": "Hubble Ring,Kizim Landing,Readdy Enterprise,Wiberg's Progress,Fermi Landing"
+ },
+ {
+ "name": "Munchep",
+ "pos_x": -41.1875,
+ "pos_y": -125.59375,
+ "pos_z": -67.21875,
+ "stations": "Fernao do Po Terminal,Tavernier Platform,Skolem Depot"
+ },
+ {
+ "name": "Mundandjabe",
+ "pos_x": 150.1875,
+ "pos_y": -33.6875,
+ "pos_z": 29.21875,
+ "stations": "Cheranovsky Settlement,Chernykh Port,Westphal Station"
+ },
+ {
+ "name": "Mundigal",
+ "pos_x": 28.1875,
+ "pos_y": -123.15625,
+ "pos_z": -89.4375,
+ "stations": "Fukushima Vision,Henry Orbital,Fox Orbital,Pogson Arsenal"
+ },
+ {
+ "name": "Mundii",
+ "pos_x": 85.5625,
+ "pos_y": -1.0625,
+ "pos_z": -0.375,
+ "stations": "Walker Terminal,Elder Hub,Birmingham Prospect"
+ },
+ {
+ "name": "Mundindja",
+ "pos_x": 140.75,
+ "pos_y": 75.8125,
+ "pos_z": -63.9375,
+ "stations": "Nikitin Outpost"
+ },
+ {
+ "name": "Mundine",
+ "pos_x": 143.59375,
+ "pos_y": -92.71875,
+ "pos_z": 19.25,
+ "stations": "Metcalf Settlement,Mourelle Reach,Jones Prospect"
+ },
+ {
+ "name": "Mundjiga",
+ "pos_x": 32.75,
+ "pos_y": -95.625,
+ "pos_z": 32.53125,
+ "stations": "Sagan Port,Zahn Survey"
+ },
+ {
+ "name": "Mundumnonne",
+ "pos_x": 45.59375,
+ "pos_y": -59.25,
+ "pos_z": -92.71875,
+ "stations": "Elgin Settlement,Crown Point"
+ },
+ {
+ "name": "Munfayl",
+ "pos_x": -24.15625,
+ "pos_y": -86.15625,
+ "pos_z": 2.53125,
+ "stations": "Samson,Nicolet Orbital,Bondarek Orbital,Gaultier de Varennes Hub"
+ },
+ {
+ "name": "Munshin",
+ "pos_x": 101.65625,
+ "pos_y": -111.3125,
+ "pos_z": 39.1875,
+ "stations": "Bessel Dock,Sterny's Refuge,Ocrinox's Orbiter,Goonan Installation,Harding Station,Brundage Vision,Herreshoff's Pride,Humphreys Survey"
+ },
+ {
+ "name": "Muraba",
+ "pos_x": -59.09375,
+ "pos_y": -14.875,
+ "pos_z": -101.09375,
+ "stations": "Noakes Hub,Thiele Beacon,Bethe's Claim"
+ },
+ {
+ "name": "Muracing",
+ "pos_x": -78.6875,
+ "pos_y": 79.4375,
+ "pos_z": 64.75,
+ "stations": "Herzfeld Gateway,Bethe Station,Babbage Horizons"
+ },
+ {
+ "name": "Murama",
+ "pos_x": 115.625,
+ "pos_y": 43.8125,
+ "pos_z": -30.5625,
+ "stations": "Skvortsov Orbital,Buckell Relay"
+ },
+ {
+ "name": "Murare",
+ "pos_x": 121.6875,
+ "pos_y": -111.75,
+ "pos_z": -32.71875,
+ "stations": "Schmidt Orbital,Anastase Perrotin Terminal,Binney Hub,Resnick Plant,Lefschetz Installation"
+ },
+ {
+ "name": "Murarija",
+ "pos_x": -73.28125,
+ "pos_y": 87.90625,
+ "pos_z": 87.125,
+ "stations": "Prunariu Platform"
+ },
+ {
+ "name": "Muras",
+ "pos_x": 107.375,
+ "pos_y": 43.78125,
+ "pos_z": 50.4375,
+ "stations": "Glazkov Mine"
+ },
+ {
+ "name": "Murato",
+ "pos_x": 91.53125,
+ "pos_y": -99.53125,
+ "pos_z": 66.5625,
+ "stations": "Tietjen Ring,Encke Ring"
+ },
+ {
+ "name": "Murawal",
+ "pos_x": 105.4375,
+ "pos_y": -122.34375,
+ "pos_z": 105.375,
+ "stations": "Yize Vision,Lalande Vision,Regiomontanus Station"
+ },
+ {
+ "name": "Muri",
+ "pos_x": -134.46875,
+ "pos_y": 4.28125,
+ "pos_z": -10.625,
+ "stations": "Vasquez de Coronado Dock,Gibson Landing,Maler Colony"
+ },
+ {
+ "name": "Muriges",
+ "pos_x": 4.5,
+ "pos_y": -81.5,
+ "pos_z": 1.46875,
+ "stations": "Scully-Power Hangar,Wegener Holdings,Grechko Plant"
+ },
+ {
+ "name": "Murnako",
+ "pos_x": 77.34375,
+ "pos_y": -138.71875,
+ "pos_z": 25.9375,
+ "stations": "Mallory Base,Hogg Terminal,Hencke Survey,Arnold Enterprise"
+ },
+ {
+ "name": "Murnakum",
+ "pos_x": -69.9375,
+ "pos_y": -57.59375,
+ "pos_z": -12.15625,
+ "stations": "Manakov Port,Appel Hub,Braun Enterprise,Christopher Relay,Rand Hub"
+ },
+ {
+ "name": "Murni",
+ "pos_x": 76,
+ "pos_y": -32.71875,
+ "pos_z": -94.90625,
+ "stations": "Lichtenberg Colony"
+ },
+ {
+ "name": "Muruidon",
+ "pos_x": -43.8125,
+ "pos_y": -74.625,
+ "pos_z": 69.0625,
+ "stations": "Crippen Orbital,Drexler Station,Daimler Gateway"
+ },
+ {
+ "name": "Muruidooges",
+ "pos_x": 164.25,
+ "pos_y": -91.15625,
+ "pos_z": -41.84375,
+ "stations": "Emshwiller Hub,Sternbach Vision,Turner Vision"
+ },
+ {
+ "name": "Murunderet",
+ "pos_x": -40.75,
+ "pos_y": 16.125,
+ "pos_z": 141.375,
+ "stations": "Nesvadba Dock,Merle Station,Thompson Landing"
+ },
+ {
+ "name": "Murung",
+ "pos_x": -31.5,
+ "pos_y": 23.6875,
+ "pos_z": 75.9375,
+ "stations": "Strzelecki Orbital,Huberath Hub,Napier Gateway,Verrazzano's Inheritance"
+ },
+ {
+ "name": "Murung Kuna",
+ "pos_x": -100.4375,
+ "pos_y": -25.21875,
+ "pos_z": -54.34375,
+ "stations": "Paulmier de Gonneville Beacon"
+ },
+ {
+ "name": "Murungh",
+ "pos_x": -12.84375,
+ "pos_y": -42.125,
+ "pos_z": 7.5625,
+ "stations": "Wohler's Folly,Weston Plant,Midgeley Port"
+ },
+ {
+ "name": "Murus",
+ "pos_x": 93.4375,
+ "pos_y": -103.40625,
+ "pos_z": 5.9375,
+ "stations": "Bradshaw Penal colony,Stephan Platform,Schmidt Vision"
+ },
+ {
+ "name": "Murusa",
+ "pos_x": -78.8125,
+ "pos_y": -47.09375,
+ "pos_z": 113.46875,
+ "stations": "Birkhoff Works,Wells Legacy"
+ },
+ {
+ "name": "Muspell",
+ "pos_x": 62.34375,
+ "pos_y": -64.875,
+ "pos_z": 49.15625,
+ "stations": "Vercors Lab,Nelson Landing"
+ },
+ {
+ "name": "Muss",
+ "pos_x": 30.25,
+ "pos_y": -97.96875,
+ "pos_z": 119.875,
+ "stations": "Zhuravleva Holdings,Vaucouleurs Point"
+ },
+ {
+ "name": "Mussche",
+ "pos_x": 154.09375,
+ "pos_y": 38.9375,
+ "pos_z": 73.40625,
+ "stations": "Disch Relay"
+ },
+ {
+ "name": "Musscheim",
+ "pos_x": 117.90625,
+ "pos_y": 95.46875,
+ "pos_z": 60.875,
+ "stations": "Herreshoff Relay,Bethke Hangar,Cousin's Progress"
+ },
+ {
+ "name": "Musubia",
+ "pos_x": 12.78125,
+ "pos_y": 113.78125,
+ "pos_z": 66.9375,
+ "stations": "Przhevalsky Ring,Kovalevskaya Dock,Williams Port"
+ },
+ {
+ "name": "Muth",
+ "pos_x": 41,
+ "pos_y": -30.59375,
+ "pos_z": -19,
+ "stations": "Gutierrez Hub,al-Khowarizmi Keep"
+ },
+ {
+ "name": "Muthi",
+ "pos_x": 126.65625,
+ "pos_y": 8.875,
+ "pos_z": 3.3125,
+ "stations": "Buffett City,Gerrold Depot,Bamford Plant"
+ },
+ {
+ "name": "Muthia",
+ "pos_x": 205.59375,
+ "pos_y": -170.875,
+ "pos_z": 70.90625,
+ "stations": "Kosai Colony"
+ },
+ {
+ "name": "Muthnir",
+ "pos_x": 184.75,
+ "pos_y": -176.6875,
+ "pos_z": 87.375,
+ "stations": "Hughes Holdings,Schommer Survey,Takahashi's Pride"
+ },
+ {
+ "name": "Muthniu",
+ "pos_x": 71.84375,
+ "pos_y": 57.59375,
+ "pos_z": -38.03125,
+ "stations": "Behnken Orbital,Chargaff Ring,Moisuc Station,Crown Landing"
+ },
+ {
+ "name": "Mutj",
+ "pos_x": 16.34375,
+ "pos_y": -23.5,
+ "pos_z": 61.25,
+ "stations": "Watts Ring,Bunnell Enterprise"
+ },
+ {
+ "name": "Mutjarabi",
+ "pos_x": 101.25,
+ "pos_y": -84.3125,
+ "pos_z": -62.5625,
+ "stations": "Bok Hub,Dantec Depot"
+ },
+ {
+ "name": "Mutpuri",
+ "pos_x": 88.0625,
+ "pos_y": -172.8125,
+ "pos_z": 136.71875,
+ "stations": "Kopal Terminal,Helin Mines,Finlay Enterprise,Tapinas Keep"
+ },
+ {
+ "name": "Mutujiali",
+ "pos_x": 20.8125,
+ "pos_y": -83.4375,
+ "pos_z": 31.9375,
+ "stations": "Siegel Orbital,Hirayama Port,Araki Station,Webb Base,Shirley Terminal"
+ },
+ {
+ "name": "Mutumu",
+ "pos_x": -35.5625,
+ "pos_y": 87.34375,
+ "pos_z": -66.71875,
+ "stations": "Locke Station,Nielsen Holdings,Ross Port"
+ },
+ {
+ "name": "Mututna",
+ "pos_x": -49.1875,
+ "pos_y": 129.28125,
+ "pos_z": 59.875,
+ "stations": "Lubin Refinery,Newton Horizons"
+ },
+ {
+ "name": "Mututnahpu",
+ "pos_x": -141.375,
+ "pos_y": -83,
+ "pos_z": 6.625,
+ "stations": "Farouk Orbital"
+ },
+ {
+ "name": "MV Virginis",
+ "pos_x": 34.6875,
+ "pos_y": 66.46875,
+ "pos_z": 63.71875,
+ "stations": "Weitz Hub,McMonagle Horizons,Behnken Orbital,Grimwood Relay,Bellamy Horizons"
+ },
+ {
+ "name": "Mvubandowag",
+ "pos_x": -138.125,
+ "pos_y": 10.125,
+ "pos_z": 73.875,
+ "stations": "Teng-hui Port,Alexandria Hub,Read Relay,Frimout Port,Palmer Works"
+ },
+ {
+ "name": "Mwamba",
+ "pos_x": -51.9375,
+ "pos_y": -45,
+ "pos_z": -42.5,
+ "stations": "Vishweswarayya Dock"
+ },
+ {
+ "name": "Mwambe",
+ "pos_x": 118.53125,
+ "pos_y": -182.09375,
+ "pos_z": 92.09375,
+ "stations": "Furukawa Base,Krenkel Landing,Plaskett Dock"
+ },
+ {
+ "name": "Mwamen",
+ "pos_x": -6.125,
+ "pos_y": -88.65625,
+ "pos_z": -15.59375,
+ "stations": "Shunkai Orbital,Chernykh Port,Frimout Hub,Chebyshev Relay"
+ },
+ {
+ "name": "Mwamitoto",
+ "pos_x": -9.25,
+ "pos_y": 74.8125,
+ "pos_z": -82.1875,
+ "stations": "Pippin Enterprise,Palmer Port,Davis Point"
+ },
+ {
+ "name": "MY Apodis",
+ "pos_x": 56,
+ "pos_y": -24.4375,
+ "pos_z": 41.9375,
+ "stations": "Crossfield Stop"
+ },
+ {
+ "name": "Myrbat",
+ "pos_x": -63.53125,
+ "pos_y": -53.28125,
+ "pos_z": -23.25,
+ "stations": "Camarda Enterprise,Lee Enterprise,de Sousa Survey,Rupak K Paul,Myrbat Station"
+ },
+ {
+ "name": "MZ Ursae Majoris",
+ "pos_x": -31.28125,
+ "pos_y": 87.65625,
+ "pos_z": -31.71875,
+ "stations": "Tenn Landing,Collins Settlement,Lawhead Bastion"
+ },
+ {
+ "name": "n Puppis",
+ "pos_x": 88.4375,
+ "pos_y": -2.4375,
+ "pos_z": -53.40625,
+ "stations": "Gresh City,Christian Keep,Rosenberg Installation,Bisson Settlement"
+ },
+ {
+ "name": "Na Chac Og",
+ "pos_x": 78.09375,
+ "pos_y": -21.125,
+ "pos_z": -124.75,
+ "stations": "Temple Terminal,Marusek Enterprise,Leinster Landing,Larson Station,Darnielle Barracks"
+ },
+ {
+ "name": "Na Chem",
+ "pos_x": 3.34375,
+ "pos_y": 64.03125,
+ "pos_z": 76.21875,
+ "stations": "Rorschach Horizons,Kuhn Installation,Melroy Dock"
+ },
+ {
+ "name": "Na Chipini",
+ "pos_x": 34.5,
+ "pos_y": -107.90625,
+ "pos_z": 133.75,
+ "stations": "Herschel Oasis,Crown Platform"
+ },
+ {
+ "name": "Na Chun Di",
+ "pos_x": -67.40625,
+ "pos_y": 135.8125,
+ "pos_z": 37.09375,
+ "stations": "Schouten Gateway,Dummer Terminal,Hand Keep"
+ },
+ {
+ "name": "Na Zha",
+ "pos_x": 165.84375,
+ "pos_y": 3.75,
+ "pos_z": -75.125,
+ "stations": "Rose Ring,Malocello Silo"
+ },
+ {
+ "name": "Na Zotzil",
+ "pos_x": -133.125,
+ "pos_y": 51.6875,
+ "pos_z": 19.15625,
+ "stations": "Lyakhov Terminal,Hubble Outpost,Tem Landing"
+ },
+ {
+ "name": "Nabatean",
+ "pos_x": 23.125,
+ "pos_y": -66.28125,
+ "pos_z": 22.46875,
+ "stations": "Vishweswarayya Orbital,George Beacon,Ellis Gateway,Noriega City,Trevithick Beacon"
+ },
+ {
+ "name": "Naduninda",
+ "pos_x": 145.90625,
+ "pos_y": 18.5,
+ "pos_z": 72.1875,
+ "stations": "Robson Mines,Dyr Enterprise,Caryanda Enterprise,Konscak's Folly"
+ },
+ {
+ "name": "Nadunuvii",
+ "pos_x": 29.25,
+ "pos_y": 107.90625,
+ "pos_z": 69.46875,
+ "stations": "Wrangell Station,Dirac Landing,Gurney Platform,Maybury Refinery"
+ },
+ {
+ "name": "Nadur",
+ "pos_x": 115.8125,
+ "pos_y": -14.15625,
+ "pos_z": 126.5,
+ "stations": "Deere Barracks,Longyear Landing,Sturt Freeport"
+ },
+ {
+ "name": "Naduscapati",
+ "pos_x": 128.1875,
+ "pos_y": 19.84375,
+ "pos_z": 49.0625,
+ "stations": "Ozanne Colony,Bischoff Depot,McAllaster Penal colony"
+ },
+ {
+ "name": "Naduvul",
+ "pos_x": 0.53125,
+ "pos_y": -67.5625,
+ "pos_z": 64.5625,
+ "stations": "Clifford Hub,Ramsay's Claim"
+ },
+ {
+ "name": "Naduwal",
+ "pos_x": 57.0625,
+ "pos_y": -15,
+ "pos_z": -128.34375,
+ "stations": "Sabine Beacon,Slusser Settlement,Acaba Barracks"
+ },
+ {
+ "name": "Naebin",
+ "pos_x": 124.1875,
+ "pos_y": 41.375,
+ "pos_z": -47.34375,
+ "stations": "Archambault Orbital"
+ },
+ {
+ "name": "Naebing",
+ "pos_x": 108.71875,
+ "pos_y": -214.15625,
+ "pos_z": -3.5625,
+ "stations": "Nakano Beacon"
+ },
+ {
+ "name": "Naebisaako",
+ "pos_x": 25.96875,
+ "pos_y": -27.5,
+ "pos_z": 88.09375,
+ "stations": "Rushd Gateway,Cayley Hub,Gaultier de Varennes Hub,Ericsson Orbital"
+ },
+ {
+ "name": "Naebisoci",
+ "pos_x": -40.8125,
+ "pos_y": -140.78125,
+ "pos_z": 120.84375,
+ "stations": "Crown Colony"
+ },
+ {
+ "name": "Naebisulfr",
+ "pos_x": 101.78125,
+ "pos_y": -40.90625,
+ "pos_z": 34.09375,
+ "stations": "Dhawan Dock,Geston Observatory,Thesiger Penal colony"
+ },
+ {
+ "name": "Naebitici",
+ "pos_x": 102.40625,
+ "pos_y": -133.15625,
+ "pos_z": -78.75,
+ "stations": "Taylor Jr. Reformatory,Dhawan Point"
+ },
+ {
+ "name": "Nagages",
+ "pos_x": 151.25,
+ "pos_y": -64.03125,
+ "pos_z": 2.96875,
+ "stations": "Giacobini Estate,Kirk's Progress,Gurney Prospect"
+ },
+ {
+ "name": "Naganjin",
+ "pos_x": 52.5,
+ "pos_y": -172.34375,
+ "pos_z": 142.90625,
+ "stations": "Flagg Station,Oja Ring"
+ },
+ {
+ "name": "Nagans",
+ "pos_x": 37.1875,
+ "pos_y": -109.90625,
+ "pos_z": -52.1875,
+ "stations": "Stephan Platform"
+ },
+ {
+ "name": "Nagarans",
+ "pos_x": -48.84375,
+ "pos_y": -71.375,
+ "pos_z": -39.15625,
+ "stations": "Sevastyanov Survey,Shukor Mines"
+ },
+ {
+ "name": "Nagarlaw",
+ "pos_x": 16.25,
+ "pos_y": 40.40625,
+ "pos_z": 72.75,
+ "stations": "Crown Dock"
+ },
+ {
+ "name": "Nagaru",
+ "pos_x": -116.34375,
+ "pos_y": 12.5,
+ "pos_z": 100.34375,
+ "stations": "Slusser Platform"
+ },
+ {
+ "name": "Nagasairu",
+ "pos_x": 34.78125,
+ "pos_y": -8.375,
+ "pos_z": 77.84375,
+ "stations": "Teng-hui Station,Efremov Works,Ejeta Dock,Ansari Station,Garriott Beacon"
+ },
+ {
+ "name": "Nagaybaks",
+ "pos_x": 35.0625,
+ "pos_y": -48,
+ "pos_z": 70.75,
+ "stations": "Noether Penal colony,Julian Hub,Collins Terminal,Johnson Dock,Borlaug Keep"
+ },
+ {
+ "name": "Nagaybuwa",
+ "pos_x": -6.8125,
+ "pos_y": -47.5625,
+ "pos_z": 113.0625,
+ "stations": "Libby Port,Higginbotham Port,Viktorenko Dock,Priestley Keep"
+ },
+ {
+ "name": "Nageni",
+ "pos_x": -35.84375,
+ "pos_y": -153.6875,
+ "pos_z": 18,
+ "stations": "Courvoisier Station,Kibalchich Port,Bradfield Terminal,Martin Base"
+ },
+ {
+ "name": "Nagi",
+ "pos_x": 127.625,
+ "pos_y": -105.5625,
+ "pos_z": -53.15625,
+ "stations": "Bolkow City,Rizvi Station,Roth Dock,Legendre Horizons,Champlain Bastion"
+ },
+ {
+ "name": "Nagii",
+ "pos_x": -55.34375,
+ "pos_y": 38.25,
+ "pos_z": -158.75,
+ "stations": "Maclaurin Vision,Hillary Hub,Swanwick Station,Bond Station,He Terminal"
+ },
+ {
+ "name": "Nagnaiwae",
+ "pos_x": 6.0625,
+ "pos_y": 15.9375,
+ "pos_z": 152.46875,
+ "stations": "Quimper Ring,Jacobi Vision,Penn Beacon"
+ },
+ {
+ "name": "Nagnatae",
+ "pos_x": 35.46875,
+ "pos_y": 8.8125,
+ "pos_z": 42.15625,
+ "stations": "Howard City,Cartwright City,Kondakova Port"
+ },
+ {
+ "name": "Nagni",
+ "pos_x": 85.21875,
+ "pos_y": -253,
+ "pos_z": 15.46875,
+ "stations": "Naboth Terminal,Myasishchev Terminal"
+ },
+ {
+ "name": "Nagnutli",
+ "pos_x": 144.78125,
+ "pos_y": -20.15625,
+ "pos_z": 46.90625,
+ "stations": "Smith Landing,Hammond Platform,Franklin Terminal,Wyndham Installation,Behring Dock"
+ },
+ {
+ "name": "Nagybold",
+ "pos_x": 47.5,
+ "pos_y": 86.34375,
+ "pos_z": 13.09375,
+ "stations": "Poleshchuk Platform,Gordon Hangar,Webb Enterprise"
+ },
+ {
+ "name": "Nahua",
+ "pos_x": 27.71875,
+ "pos_y": -119.625,
+ "pos_z": 45.625,
+ "stations": "Fuller Vision,Hodgson Camp,Williamson Depot"
+ },
+ {
+ "name": "Nahuahini",
+ "pos_x": 53.84375,
+ "pos_y": -248.84375,
+ "pos_z": 78.65625,
+ "stations": "Plait Vision,Beg Terminal"
+ },
+ {
+ "name": "Nahuaru",
+ "pos_x": 46.34375,
+ "pos_y": 7.5,
+ "pos_z": 143.59375,
+ "stations": "Williams Vision,Collins Gateway"
+ },
+ {
+ "name": "Nahuatl",
+ "pos_x": 65.25,
+ "pos_y": -85.75,
+ "pos_z": -6.15625,
+ "stations": "Kowal Terminal,Davis Relay,Fernandes de Queiros Camp,Artzybasheff Terminal,Roth Port,Zhukovsky Enterprise,Van Scyoc Vista,Ritchey Station,Burnham Orbital,Plait Hub,Rigaux Vision,Polikarpov Dock,Robert Vista,Smith Forum,Lyne Terminal"
+ },
+ {
+ "name": "Nahumla",
+ "pos_x": -57.59375,
+ "pos_y": -1.40625,
+ "pos_z": 103.28125,
+ "stations": "Malenchenko Settlement,Poleshchuk Holdings"
+ },
+ {
+ "name": "Nahundi",
+ "pos_x": 28,
+ "pos_y": 47.15625,
+ "pos_z": -13.28125,
+ "stations": "Nelson Landing,Boodt Installation,Bella Station,Bouch Survey"
+ },
+ {
+ "name": "Nai Hou Jiu",
+ "pos_x": 85.34375,
+ "pos_y": -170.96875,
+ "pos_z": 141.71875,
+ "stations": "Hamilton's Folly,Esclangon Enterprise,Krigstein Terminal"
+ },
+ {
+ "name": "Nai No Kami",
+ "pos_x": 133.125,
+ "pos_y": -244.46875,
+ "pos_z": 110.84375,
+ "stations": "Halley Gateway"
+ },
+ {
+ "name": "Nai Nones",
+ "pos_x": -40.90625,
+ "pos_y": 30.96875,
+ "pos_z": -165.0625,
+ "stations": "Brust Terminal,Perez Platform,Lawhead Horizons"
+ },
+ {
+ "name": "Nai Shoro",
+ "pos_x": 53.15625,
+ "pos_y": -145.25,
+ "pos_z": -38.71875,
+ "stations": "Gaughan Survey,Wendelin Relay"
+ },
+ {
+ "name": "Naik",
+ "pos_x": 63.4375,
+ "pos_y": 108.46875,
+ "pos_z": 7.53125,
+ "stations": "Koontz Terminal,Rocklynne Port,Erdos Enterprise"
+ },
+ {
+ "name": "Naika",
+ "pos_x": 116.34375,
+ "pos_y": -174.34375,
+ "pos_z": 129.40625,
+ "stations": "Antoniadi Hub,Auzout Port,Shapley Settlement,Webb Point"
+ },
+ {
+ "name": "Naikun",
+ "pos_x": 184.25,
+ "pos_y": -116.59375,
+ "pos_z": 63.625,
+ "stations": "Oshima Orbital,Grant Exchange"
+ },
+ {
+ "name": "Nait",
+ "pos_x": 131.25,
+ "pos_y": -20.4375,
+ "pos_z": -70.3125,
+ "stations": "Faris Hub,Makeev Base,Cartwright Barracks"
+ },
+ {
+ "name": "Naita",
+ "pos_x": -17.6875,
+ "pos_y": 44.3125,
+ "pos_z": 117.625,
+ "stations": "Whitson Colony,Bennett Camp"
+ },
+ {
+ "name": "Naiti",
+ "pos_x": 24.5,
+ "pos_y": -13.65625,
+ "pos_z": -76.625,
+ "stations": "Fremont Keep,Deluc Settlement"
+ },
+ {
+ "name": "Naitis",
+ "pos_x": 39.9375,
+ "pos_y": 77.75,
+ "pos_z": 21.875,
+ "stations": "Ford Dock,Deluc Keep,Okorafor Dock,Eckford Prospect"
+ },
+ {
+ "name": "Naits",
+ "pos_x": -59.625,
+ "pos_y": -42.5625,
+ "pos_z": 20.3125,
+ "stations": "McDivitt Orbital"
+ },
+ {
+ "name": "Nakako",
+ "pos_x": -95.5,
+ "pos_y": 79.65625,
+ "pos_z": -38.1875,
+ "stations": "Peano Landing,Szentmartony Landing,Foale Penal colony"
+ },
+ {
+ "name": "Nakap",
+ "pos_x": -73.75,
+ "pos_y": -31.03125,
+ "pos_z": 41.75,
+ "stations": "George Terminal,Farrukh Base"
+ },
+ {
+ "name": "Nakaruwuti",
+ "pos_x": 148.65625,
+ "pos_y": -172.625,
+ "pos_z": 75.625,
+ "stations": "Gustafsson Prospect,Bohme Terminal,Barmin Vision,Arnold Schwassmann Beacon"
+ },
+ {
+ "name": "Nakau",
+ "pos_x": 137.96875,
+ "pos_y": -9.875,
+ "pos_z": -14.09375,
+ "stations": "Vyssotsky Platform"
+ },
+ {
+ "name": "Nakula",
+ "pos_x": 80.65625,
+ "pos_y": 98.59375,
+ "pos_z": 29.5625,
+ "stations": "Fisher Port,Effinger Town,Crouch Port,Macarthur Enterprise,Sherrington's Progress"
+ },
+ {
+ "name": "Nakulha",
+ "pos_x": -24.875,
+ "pos_y": -9.5625,
+ "pos_z": 96,
+ "stations": "Vance Enterprise,Tucker City,Fossum Vision,Taylor Hub"
+ },
+ {
+ "name": "Nakuma",
+ "pos_x": -154.59375,
+ "pos_y": 34.1875,
+ "pos_z": -18.75,
+ "stations": "Ivanchenkov Mines,Lopez-Alegria Outpost"
+ },
+ {
+ "name": "Nal Yax",
+ "pos_x": -19.1875,
+ "pos_y": -161.15625,
+ "pos_z": 2.53125,
+ "stations": "Farghani Hub"
+ },
+ {
+ "name": "Nal Yeh",
+ "pos_x": 7.25,
+ "pos_y": -3.125,
+ "pos_z": 54.65625,
+ "stations": "Karl Diesel Installation"
+ },
+ {
+ "name": "Nalu",
+ "pos_x": 39.0625,
+ "pos_y": 57.71875,
+ "pos_z": 154.3125,
+ "stations": "Yaping Settlement,Oppenheimer Beacon"
+ },
+ {
+ "name": "Namab",
+ "pos_x": -36.4375,
+ "pos_y": -38.78125,
+ "pos_z": 80.625,
+ "stations": "Pytheas Settlement,Shepard Dock"
+ },
+ {
+ "name": "Namago",
+ "pos_x": 100.1875,
+ "pos_y": -32.125,
+ "pos_z": -143.21875,
+ "stations": "Leckie Settlement,Hirase Mine"
+ },
+ {
+ "name": "Namai",
+ "pos_x": 120.875,
+ "pos_y": 39.5,
+ "pos_z": 14.5,
+ "stations": "Behnken Dock,Libby Camp,Qian Enterprise"
+ },
+ {
+ "name": "Namaka",
+ "pos_x": -25.125,
+ "pos_y": 86.0625,
+ "pos_z": 3.15625,
+ "stations": "Cantor Platform,Erikson Settlement,Clayton Mines,Dozois Beacon,Atwood Settlement"
+ },
+ {
+ "name": "Namamasairu",
+ "pos_x": 157.40625,
+ "pos_y": -73.65625,
+ "pos_z": -1.5,
+ "stations": "Ptack Station,Sawyer Base,Fujikawa Ring,Chernykh Ring"
+ },
+ {
+ "name": "Namarii",
+ "pos_x": 67.3125,
+ "pos_y": -120.8125,
+ "pos_z": -20.53125,
+ "stations": "Goldberg Station,Hughes Hub,Joy Terminal,Czerneda Survey"
+ },
+ {
+ "name": "Namasa",
+ "pos_x": 99.03125,
+ "pos_y": -116.71875,
+ "pos_z": 164.5,
+ "stations": "Schumacher Vision,Israel Port"
+ },
+ {
+ "name": "Namat",
+ "pos_x": 55.75,
+ "pos_y": -187.46875,
+ "pos_z": 90.21875,
+ "stations": "Goryu Terminal"
+ },
+ {
+ "name": "Namatho",
+ "pos_x": 16.65625,
+ "pos_y": -82.40625,
+ "pos_z": -62.15625,
+ "stations": "Hurley Settlement,Montrose's Progress,Ryazanski Refinery"
+ },
+ {
+ "name": "Namayu",
+ "pos_x": 90.125,
+ "pos_y": -48.125,
+ "pos_z": -118.25,
+ "stations": "Bordage Survey,Wilkes Installation,Bayley Outpost"
+ },
+ {
+ "name": "Namba",
+ "pos_x": -5.125,
+ "pos_y": -154.21875,
+ "pos_z": -12.5,
+ "stations": "McCrea Estate,Watson Vision,Jaitinder Singh,Mori Platform,Lambert Plant,Pordenone Terminal,Asimov Landing"
+ },
+ {
+ "name": "Nambal",
+ "pos_x": 100.46875,
+ "pos_y": -128.90625,
+ "pos_z": -0.40625,
+ "stations": "Gabrielli Landing,Lewis Station,Jung Orbital,Mitchell Beacon,Mizuno Terminal,Neff Survey,Townes Port"
+ },
+ {
+ "name": "Nambi",
+ "pos_x": -6.6875,
+ "pos_y": -211.75,
+ "pos_z": 134.875,
+ "stations": "Hornoch Platform"
+ },
+ {
+ "name": "Nambikuara",
+ "pos_x": -88.0625,
+ "pos_y": -14.65625,
+ "pos_z": 75.96875,
+ "stations": "Danvers Station,Schade Ring,Humphreys City,Navigator Terminal,Shirley City,Lukyanenko Terminal,Alexander Station,Kent Survey,Monge Holdings,Palmer Holdings"
+ },
+ {
+ "name": "Nammu",
+ "pos_x": -67.28125,
+ "pos_y": -11.3125,
+ "pos_z": 3.09375,
+ "stations": "Romanenko Horizons,Sherrington Camp"
+ },
+ {
+ "name": "Namnetes",
+ "pos_x": -64.09375,
+ "pos_y": -85.9375,
+ "pos_z": -35.4375,
+ "stations": "Monge Horizons,Fernao do Po Installation,Jolliet Enterprise,Stephenson Landing,Rose Vision"
+ },
+ {
+ "name": "Namniez",
+ "pos_x": 97.3125,
+ "pos_y": -162.4375,
+ "pos_z": 109.8125,
+ "stations": "Frost Depot,Pons Dock,South Station,Hayashi Hub,Yakovlev Port,Nouvel Ring,Chandra Vision,Izumikawa Station,Shklovsky Orbital,Ledyard Horizons,Silverberg Laboratory"
+ },
+ {
+ "name": "Namo",
+ "pos_x": 111.0625,
+ "pos_y": -117.40625,
+ "pos_z": 143.28125,
+ "stations": "Yakovlev Landing,O'Neill Holdings"
+ },
+ {
+ "name": "Namon",
+ "pos_x": 91.1875,
+ "pos_y": -79.1875,
+ "pos_z": 74.5625,
+ "stations": "Bailey Penal colony,Maanen Dock"
+ },
+ {
+ "name": "Namorodo",
+ "pos_x": 109.15625,
+ "pos_y": -53.53125,
+ "pos_z": -13.0625,
+ "stations": "Sevastyanov Station"
+ },
+ {
+ "name": "Namorovi",
+ "pos_x": 79.4375,
+ "pos_y": -210.125,
+ "pos_z": -39.84375,
+ "stations": "Haack's Claim"
+ },
+ {
+ "name": "Namte",
+ "pos_x": 75.03125,
+ "pos_y": 1.5,
+ "pos_z": -25.40625,
+ "stations": "Johnson Orbital,MacLean Orbital,Fung Station,McKay Settlement,Walker's Progress"
+ },
+ {
+ "name": "Nan Cruagsa",
+ "pos_x": -135.71875,
+ "pos_y": 54.625,
+ "pos_z": 39.1875,
+ "stations": "Velazquez Station"
+ },
+ {
+ "name": "Nan Hsie",
+ "pos_x": 77.125,
+ "pos_y": 3.15625,
+ "pos_z": 76.75,
+ "stations": "Tarentum Platform"
+ },
+ {
+ "name": "Nan Sapwe",
+ "pos_x": 48.3125,
+ "pos_y": -15.9375,
+ "pos_z": 40.78125,
+ "stations": "White Enterprise"
+ },
+ {
+ "name": "Nan Wera",
+ "pos_x": 76.96875,
+ "pos_y": -104.9375,
+ "pos_z": 127.46875,
+ "stations": "Pannekoek Mines,Hansteen Terminal,Miyasaka Landing,Gurevich Base"
+ },
+ {
+ "name": "Nan Yamanes",
+ "pos_x": -88.65625,
+ "pos_y": -100.125,
+ "pos_z": -70.9375,
+ "stations": "Ayerdhal Vision,Adams Silo"
+ },
+ {
+ "name": "Nana Buluku",
+ "pos_x": 116.1875,
+ "pos_y": -73.75,
+ "pos_z": -53.90625,
+ "stations": "Harding Outpost"
+ },
+ {
+ "name": "Nana De",
+ "pos_x": -25.78125,
+ "pos_y": -165,
+ "pos_z": 17.53125,
+ "stations": "Greenstein Base"
+ },
+ {
+ "name": "Nanabozho",
+ "pos_x": 22.9375,
+ "pos_y": -35.75,
+ "pos_z": 24.15625,
+ "stations": "Lamarck Orbital,Martin Silo"
+ },
+ {
+ "name": "Nanamatha",
+ "pos_x": -17.125,
+ "pos_y": 34.1875,
+ "pos_z": -103.34375,
+ "stations": "Dobrovolskiy Orbital,Artsebarsky Orbital,Dunyach Survey,Stephenson Prospect,Forbes Prospect"
+ },
+ {
+ "name": "Nanangolans",
+ "pos_x": 144.09375,
+ "pos_y": 75,
+ "pos_z": -68.5625,
+ "stations": "Felice Camp,Frobenius Camp"
+ },
+ {
+ "name": "Nanapan",
+ "pos_x": 37.625,
+ "pos_y": -200.96875,
+ "pos_z": -11.09375,
+ "stations": "Friedrich Peters Beacon"
+ },
+ {
+ "name": "Nanarilobo",
+ "pos_x": -29.625,
+ "pos_y": 157.90625,
+ "pos_z": -10.6875,
+ "stations": "Doctorow Freeport"
+ },
+ {
+ "name": "Nanata",
+ "pos_x": 120.28125,
+ "pos_y": 1.78125,
+ "pos_z": 89.59375,
+ "stations": "Mayr Station"
+ },
+ {
+ "name": "Nanatani",
+ "pos_x": 144,
+ "pos_y": -5,
+ "pos_z": 33.0625,
+ "stations": "Onnes Works,Lysenko Camp"
+ },
+ {
+ "name": "Nanatauvii",
+ "pos_x": -20.90625,
+ "pos_y": -191.375,
+ "pos_z": 46.90625,
+ "stations": "Aoki Base"
+ },
+ {
+ "name": "Nanatay",
+ "pos_x": 111.5625,
+ "pos_y": -119.84375,
+ "pos_z": 21.71875,
+ "stations": "Dornier Works"
+ },
+ {
+ "name": "Nanawi",
+ "pos_x": 74.53125,
+ "pos_y": -142.6875,
+ "pos_z": 149.59375,
+ "stations": "Porubcan Terminal,Wang Hub,Verne Beacon"
+ },
+ {
+ "name": "Nandaqui",
+ "pos_x": 25.125,
+ "pos_y": -135.65625,
+ "pos_z": -64.6875,
+ "stations": "Dassault Refinery,Wilkes Enterprise"
+ },
+ {
+ "name": "Nandarib",
+ "pos_x": 16.375,
+ "pos_y": -136.03125,
+ "pos_z": -69.34375,
+ "stations": "Potez Settlement"
+ },
+ {
+ "name": "Nandh",
+ "pos_x": 42.3125,
+ "pos_y": 7.96875,
+ "pos_z": -65.125,
+ "stations": "Nemere Terminal,Reed Gateway,Savorgnan de Brazza Lab,Wollheim Dock,Santarem Installation"
+ },
+ {
+ "name": "Nandhis",
+ "pos_x": 86.1875,
+ "pos_y": 25.21875,
+ "pos_z": -109.1875,
+ "stations": "Lowry Stop"
+ },
+ {
+ "name": "Nandhrimpo",
+ "pos_x": 153.1875,
+ "pos_y": -72.46875,
+ "pos_z": -16.71875,
+ "stations": "Fallows Point,Sleator Survey,Whelan Mine"
+ },
+ {
+ "name": "Nandhs",
+ "pos_x": 149.5,
+ "pos_y": -19.4375,
+ "pos_z": -17.09375,
+ "stations": "Lopez de Legazpi Dock,Bendell Colony,Fowler Bastion,Lethem Station,Makeev Beacon"
+ },
+ {
+ "name": "Nandigamara",
+ "pos_x": 111.46875,
+ "pos_y": -113.875,
+ "pos_z": 22.3125,
+ "stations": "Quetelet Settlement,Hill Tinsley Survey"
+ },
+ {
+ "name": "Nandinia",
+ "pos_x": 133,
+ "pos_y": -80.0625,
+ "pos_z": 35.1875,
+ "stations": "Canty Stop"
+ },
+ {
+ "name": "Nanditi",
+ "pos_x": 69.59375,
+ "pos_y": 20.40625,
+ "pos_z": 62.03125,
+ "stations": "Hale Orbital,Dampier Relay,Ferguson Gateway"
+ },
+ {
+ "name": "Nandjaba",
+ "pos_x": 128.40625,
+ "pos_y": -25.84375,
+ "pos_z": 55.53125,
+ "stations": "Rukavishnikov Plant,Malzberg Refinery,Luiken Depot,Belyayev Platform"
+ },
+ {
+ "name": "Nandjabinja",
+ "pos_x": 6.375,
+ "pos_y": -4.46875,
+ "pos_z": -63.96875,
+ "stations": "Brand City,Kregel Silo"
+ },
+ {
+ "name": "Nandjalato",
+ "pos_x": 61.5,
+ "pos_y": -2.75,
+ "pos_z": 45.125,
+ "stations": "Tereshkova Colony,Conrad Beacon,Dorsett Colony"
+ },
+ {
+ "name": "Nandjil",
+ "pos_x": 40.15625,
+ "pos_y": 43.125,
+ "pos_z": 156.65625,
+ "stations": "Gold Horizons,Guin Prospect,Stefansson Terminal,Carpini Enterprise"
+ },
+ {
+ "name": "Nandjirii",
+ "pos_x": -61.09375,
+ "pos_y": 30.25,
+ "pos_z": -19.53125,
+ "stations": "Volta Hub,Shull Settlement,Bujold Depot"
+ },
+ {
+ "name": "Nandovices",
+ "pos_x": 123,
+ "pos_y": -118.65625,
+ "pos_z": 40.25,
+ "stations": "Ohain Vision,Gaensler Silo,Nakamura Dock,Maanen Station,Aaronson Market"
+ },
+ {
+ "name": "Nang De Ti",
+ "pos_x": -150.46875,
+ "pos_y": 79.28125,
+ "pos_z": 18.46875,
+ "stations": "Yourkevitch Enterprise,Hogan Port,Bishop Orbital,Fuca Depot,Clement's Inheritance"
+ },
+ {
+ "name": "Nang Di",
+ "pos_x": 93.6875,
+ "pos_y": -77.78125,
+ "pos_z": 131,
+ "stations": "Aitken Landing,Ziegler Orbital,Matthews Orbital,Barnard Hub"
+ },
+ {
+ "name": "Nang Picori",
+ "pos_x": 53.65625,
+ "pos_y": -167.28125,
+ "pos_z": 61.375,
+ "stations": "Abetti Survey,Brooks Dock"
+ },
+ {
+ "name": "Nang Ta-khian",
+ "pos_x": -18.21875,
+ "pos_y": 26.5625,
+ "pos_z": -6.34375,
+ "stations": "Hay Point,Hadwell Orbital,Dampier Colony"
+ },
+ {
+ "name": "Nangandh",
+ "pos_x": 29.46875,
+ "pos_y": 8.53125,
+ "pos_z": 138.125,
+ "stations": "Stevin Platform,Dorsey Refinery"
+ },
+ {
+ "name": "Nanggalis",
+ "pos_x": -58.90625,
+ "pos_y": -116.5625,
+ "pos_z": -19.8125,
+ "stations": "Babbage Mine,Lopez de Haro Silo,Singer Terminal"
+ },
+ {
+ "name": "Nangkano",
+ "pos_x": -5.21875,
+ "pos_y": 96.84375,
+ "pos_z": 70.25,
+ "stations": "Sarafanov Hub,Wisoff Settlement,Kepler Orbital,Jolliet Depot"
+ },
+ {
+ "name": "Nangpo",
+ "pos_x": 3.8125,
+ "pos_y": -113.8125,
+ "pos_z": 113.21875,
+ "stations": "Lewis Hub,Dahm Platform,Suzuki Prospect,Gould Reach"
+ },
+ {
+ "name": "Nangura",
+ "pos_x": 0.78125,
+ "pos_y": -77.84375,
+ "pos_z": -81,
+ "stations": "Cabana Dock,Ahern Vista,Korzun Prospect"
+ },
+ {
+ "name": "Nangutsu",
+ "pos_x": -27.71875,
+ "pos_y": 0.4375,
+ "pos_z": 92.1875,
+ "stations": "Roosa Hub"
+ },
+ {
+ "name": "Nanka",
+ "pos_x": 108.96875,
+ "pos_y": 89.875,
+ "pos_z": -53.53125,
+ "stations": "Griffith Landing,Springer Colony,Dampier Platform"
+ },
+ {
+ "name": "Nankobi",
+ "pos_x": -58.625,
+ "pos_y": -121.75,
+ "pos_z": 25.9375,
+ "stations": "Geller Port,Deslandres Station,Arnold Platform"
+ },
+ {
+ "name": "Nankul",
+ "pos_x": -72.21875,
+ "pos_y": -55.71875,
+ "pos_z": 17.96875,
+ "stations": "Makarov Enterprise,Tombaugh Ring,Vetulani Survey,Meucci's Claim,Ackerman Arena"
+ },
+ {
+ "name": "Nann",
+ "pos_x": 117.4375,
+ "pos_y": 97.75,
+ "pos_z": 60.8125,
+ "stations": "Thiele Holdings"
+ },
+ {
+ "name": "Nanoai",
+ "pos_x": -26.96875,
+ "pos_y": -160.09375,
+ "pos_z": -41.75,
+ "stations": "Thomas Hub,Glushko Vision,Banno Hub,Franke Forum,Nelder Hub,Shaikh's Progress"
+ },
+ {
+ "name": "Nanomam",
+ "pos_x": -14.78125,
+ "pos_y": 19.65625,
+ "pos_z": -15.25,
+ "stations": "Gresley Dock,Hahn Gateway,Davis Enterprise,Carver Prospect,Savitskaya Bastion"
+ },
+ {
+ "name": "Nanoy",
+ "pos_x": 137.0625,
+ "pos_y": -14.65625,
+ "pos_z": -14.96875,
+ "stations": "Paez Ring"
+ },
+ {
+ "name": "Nanta Ti",
+ "pos_x": 122.375,
+ "pos_y": -20.21875,
+ "pos_z": 36.875,
+ "stations": "Frost Orbital,Brunner Settlement,Jones Lab"
+ },
+ {
+ "name": "Nantariates",
+ "pos_x": 79.25,
+ "pos_y": -196.3125,
+ "pos_z": 81.9375,
+ "stations": "Jenkins Dock"
+ },
+ {
+ "name": "Nanter",
+ "pos_x": -66.90625,
+ "pos_y": -29.21875,
+ "pos_z": 9.5,
+ "stations": "Bohm Plant"
+ },
+ {
+ "name": "Nantiates",
+ "pos_x": -98.21875,
+ "pos_y": -69.3125,
+ "pos_z": 50.0625,
+ "stations": "Sheckley's Inheritance,Clement Colony,J. G. Ballard Settlement,Mallett Survey"
+ },
+ {
+ "name": "Nantjangli",
+ "pos_x": 21.59375,
+ "pos_y": 93.625,
+ "pos_z": -91.03125,
+ "stations": "Reynolds Station,Larson Stop,Elcano Bastion"
+ },
+ {
+ "name": "Nanto",
+ "pos_x": -19.71875,
+ "pos_y": 78.84375,
+ "pos_z": 118.375,
+ "stations": "Morgan Orbital"
+ },
+ {
+ "name": "Nantoac",
+ "pos_x": 201.5,
+ "pos_y": -177.8125,
+ "pos_z": 46.9375,
+ "stations": "Adragna Retreat,Banno Depot"
+ },
+ {
+ "name": "Nantundan",
+ "pos_x": 110.4375,
+ "pos_y": 21.96875,
+ "pos_z": 116.3125,
+ "stations": "Helmholtz Base"
+ },
+ {
+ "name": "Napia",
+ "pos_x": 102.34375,
+ "pos_y": -124.4375,
+ "pos_z": 74.1875,
+ "stations": "Gora Terminal,Tisserand Vision,Oxley Depot,Grant Port,Fan Gateway,Bus Hub,Kowal Station,Gaughan Point,Kuchemann Port,Narlikar Dock,Petlyakov Orbital,Florine Colony,Salpeter Hub"
+ },
+ {
+ "name": "Napitic",
+ "pos_x": -99.40625,
+ "pos_y": 83.84375,
+ "pos_z": 39.90625,
+ "stations": "Serling Orbital,Cartier Prospect,Franklin Vision,Henson Prospect"
+ },
+ {
+ "name": "Naporimui",
+ "pos_x": 29.15625,
+ "pos_y": -215.5625,
+ "pos_z": 125.3125,
+ "stations": "Hewish Mines,Brashear Mines,Jacobi Arsenal"
+ },
+ {
+ "name": "Napote",
+ "pos_x": 84.15625,
+ "pos_y": -79.21875,
+ "pos_z": -40.0625,
+ "stations": "Hovgaard Asylum,Priest Point"
+ },
+ {
+ "name": "Napoyo",
+ "pos_x": 91.6875,
+ "pos_y": -146.65625,
+ "pos_z": 37.875,
+ "stations": "Rosse Camp"
+ },
+ {
+ "name": "Narai",
+ "pos_x": 88.6875,
+ "pos_y": -79.09375,
+ "pos_z": -78.375,
+ "stations": "Gernsback Port,Regiomontanus Dock,Cremona Dock,Chaviano Bastion,McDonald Plant,Francisco de Eliza Base"
+ },
+ {
+ "name": "Naraka",
+ "pos_x": 84.8125,
+ "pos_y": 132.1875,
+ "pos_z": 69.59375,
+ "stations": "Gooch Landing,Hardwick Settlement"
+ },
+ {
+ "name": "Narakapani",
+ "pos_x": 104.34375,
+ "pos_y": -93.875,
+ "pos_z": 10.03125,
+ "stations": "Leuschner Survey"
+ },
+ {
+ "name": "Narakwates",
+ "pos_x": 44.15625,
+ "pos_y": -131.59375,
+ "pos_z": 50.1875,
+ "stations": "Dubyago City,Druillet Hub,Gabriel Beacon,Kopal Works,Shen Terminal"
+ },
+ {
+ "name": "Narangga",
+ "pos_x": -82.53125,
+ "pos_y": -102.9375,
+ "pos_z": 77.71875,
+ "stations": "Eanes Dock"
+ },
+ {
+ "name": "Narans",
+ "pos_x": -135.03125,
+ "pos_y": 21.53125,
+ "pos_z": 84.5,
+ "stations": "Bondar's Claim"
+ },
+ {
+ "name": "Narantan",
+ "pos_x": 92.125,
+ "pos_y": -60.46875,
+ "pos_z": 99.59375,
+ "stations": "Takahashi Terminal,Sutcliffe Holdings,Freycinet Settlement"
+ },
+ {
+ "name": "Narasa",
+ "pos_x": -50.78125,
+ "pos_y": 22.59375,
+ "pos_z": 168.3125,
+ "stations": "Fancher Enterprise,Birmingham Mine"
+ },
+ {
+ "name": "Narasimha",
+ "pos_x": -14.25,
+ "pos_y": 1.3125,
+ "pos_z": 59.1875,
+ "stations": "Mendel Survey,Laveykin Hub,Zudov Survey"
+ },
+ {
+ "name": "Narasinha",
+ "pos_x": 19.5625,
+ "pos_y": -138.6875,
+ "pos_z": 135.34375,
+ "stations": "Giger Platform"
+ },
+ {
+ "name": "Narati Shi",
+ "pos_x": 84.9375,
+ "pos_y": 67.40625,
+ "pos_z": -107.75,
+ "stations": "Alas Colony,Salam Dock,Reed's Folly"
+ },
+ {
+ "name": "Naratones",
+ "pos_x": -77.5625,
+ "pos_y": -119.1875,
+ "pos_z": -20.84375,
+ "stations": "de Caminha Port,J. G. Ballard Port,Matthews Enterprise,Taylor Orbital,Liouville Station,Barcelos Ring,Wylie Enterprise"
+ },
+ {
+ "name": "Naraviri",
+ "pos_x": 129.53125,
+ "pos_y": -8.25,
+ "pos_z": 66.4375,
+ "stations": "Weaver Port"
+ },
+ {
+ "name": "Narbacoch",
+ "pos_x": 57.78125,
+ "pos_y": 41.28125,
+ "pos_z": 137.8125,
+ "stations": "Martins Mines,Broderick Relay"
+ },
+ {
+ "name": "Narbago",
+ "pos_x": -124.125,
+ "pos_y": 44.78125,
+ "pos_z": 82.65625,
+ "stations": "Bruce Platform,Pinto Orbital"
+ },
+ {
+ "name": "Narbudj",
+ "pos_x": 132.6875,
+ "pos_y": -20.125,
+ "pos_z": -71.6875,
+ "stations": "McMullen Camp,Pytheas Reach,Hudson Enterprise"
+ },
+ {
+ "name": "Nare",
+ "pos_x": -37.0625,
+ "pos_y": -7.1875,
+ "pos_z": -158.03125,
+ "stations": "Cabana Hub,Wheelock Orbital,Conway Mines,Fuca Penal colony"
+ },
+ {
+ "name": "Nareg",
+ "pos_x": 71.625,
+ "pos_y": -51.875,
+ "pos_z": -114.28125,
+ "stations": "Bradley Terminal,Bordage Platform"
+ },
+ {
+ "name": "Nareldi",
+ "pos_x": 10.78125,
+ "pos_y": -205.21875,
+ "pos_z": 92.375,
+ "stations": "Bessel Colony"
+ },
+ {
+ "name": "Naren",
+ "pos_x": -47.46875,
+ "pos_y": -18.78125,
+ "pos_z": -114.28125,
+ "stations": "Fisher Orbital,Jahn Station,Sekelj Beacon,Pippin Horizons,McDaniel Silo,Atwater Station,Fourier Enterprise,Kingsbury Landing,Peary Colony"
+ },
+ {
+ "name": "Nareni",
+ "pos_x": 60.09375,
+ "pos_y": 37.8125,
+ "pos_z": -71.8125,
+ "stations": "Bohm Orbital"
+ },
+ {
+ "name": "Narenses",
+ "pos_x": -1.15625,
+ "pos_y": -11.03125,
+ "pos_z": 21.875,
+ "stations": "Yang Orbital"
+ },
+ {
+ "name": "Narentes",
+ "pos_x": 75.03125,
+ "pos_y": -10.3125,
+ "pos_z": 26.1875,
+ "stations": "Boming Dock,Low Gateway"
+ },
+ {
+ "name": "Nareri",
+ "pos_x": -44.78125,
+ "pos_y": -43.78125,
+ "pos_z": 27.15625,
+ "stations": "Tapinas Installation,Grechko Beacon"
+ },
+ {
+ "name": "Nari",
+ "pos_x": -12.09375,
+ "pos_y": 170.34375,
+ "pos_z": 90.28125,
+ "stations": "Abbott Station,Metz Port,Andrews Survey"
+ },
+ {
+ "name": "Nariansan",
+ "pos_x": 38.59375,
+ "pos_y": -63.9375,
+ "pos_z": 107.15625,
+ "stations": "Carlisle Port,Burkin Hub,Faber Works,Schroeder Dock,Birkhoff Base"
+ },
+ {
+ "name": "Narim",
+ "pos_x": 142.65625,
+ "pos_y": -207.4375,
+ "pos_z": -54.25,
+ "stations": "Bisson Works,Pond Vision,Hamuy Colony"
+ },
+ {
+ "name": "Narina",
+ "pos_x": 59.125,
+ "pos_y": -209.34375,
+ "pos_z": -17.65625,
+ "stations": "Canty Colony,Eilenberg Silo"
+ },
+ {
+ "name": "Nariya",
+ "pos_x": 105.40625,
+ "pos_y": 22.0625,
+ "pos_z": 75.09375,
+ "stations": "Perry Platform,Nearchus Prospect,Shaver Orbital"
+ },
+ {
+ "name": "Nariyot",
+ "pos_x": 141,
+ "pos_y": -160.6875,
+ "pos_z": 101.21875,
+ "stations": "Searle Gateway,Yamamoto Silo,Mattingly Lab"
+ },
+ {
+ "name": "Narkinnaq",
+ "pos_x": -111.625,
+ "pos_y": 74.5625,
+ "pos_z": 55.40625,
+ "stations": "Utley Dock,Stefansson Horizons,Darnielle Installation,Navigator's Progress"
+ },
+ {
+ "name": "Narkinyin",
+ "pos_x": 100.71875,
+ "pos_y": 129.59375,
+ "pos_z": 77.40625,
+ "stations": "Volkov Settlement"
+ },
+ {
+ "name": "Narri",
+ "pos_x": -63.15625,
+ "pos_y": -80.9375,
+ "pos_z": -23.125,
+ "stations": "Bobko City,Stafford Landing,Lockhart Survey,Cabral Hub,Veblen Camp"
+ },
+ {
+ "name": "Narverdulli",
+ "pos_x": 30,
+ "pos_y": -45.4375,
+ "pos_z": -75.21875,
+ "stations": "Vela City,Revin Point"
+ },
+ {
+ "name": "Narvernaca",
+ "pos_x": -21.15625,
+ "pos_y": -150.625,
+ "pos_z": 107.4375,
+ "stations": "Saha's Inheritance"
+ },
+ {
+ "name": "Narvert",
+ "pos_x": -112.40625,
+ "pos_y": -67.96875,
+ "pos_z": -86.53125,
+ "stations": "Glidden Enterprise,Shuttleworth Station,Stirling Prospect"
+ },
+ {
+ "name": "Narveti",
+ "pos_x": 153.1875,
+ "pos_y": -206.1875,
+ "pos_z": -9.5,
+ "stations": "Robert Camp"
+ },
+ {
+ "name": "Nasaraudi",
+ "pos_x": 65.25,
+ "pos_y": -117.21875,
+ "pos_z": -45.375,
+ "stations": "Rodrigues Refinery"
+ },
+ {
+ "name": "Nashira",
+ "pos_x": -66.0625,
+ "pos_y": -111.4375,
+ "pos_z": 88.78125,
+ "stations": "Lubin Orbital,Clement Holdings,Ejeta City"
+ },
+ {
+ "name": "Nasiaverni",
+ "pos_x": 100.5,
+ "pos_y": 83.9375,
+ "pos_z": 24.03125,
+ "stations": "Marshburn Station,Varthema Penal colony,Al-Jazari Orbital,Tokubei Terminal"
+ },
+ {
+ "name": "Naso Kichi",
+ "pos_x": -5.21875,
+ "pos_y": -107.96875,
+ "pos_z": 103.125,
+ "stations": "Shaw Asylum,Elbakyan Survey"
+ },
+ {
+ "name": "Nasoda",
+ "pos_x": -83.3125,
+ "pos_y": -112.5,
+ "pos_z": -63.8125,
+ "stations": "Langford Hub"
+ },
+ {
+ "name": "Nastrond",
+ "pos_x": -75.34375,
+ "pos_y": -40.4375,
+ "pos_z": -56.75,
+ "stations": "Ardern Hill,Weierstrass Base,Rochon Ring"
+ },
+ {
+ "name": "Nat9481",
+ "pos_x": 29.09375,
+ "pos_y": -41.75,
+ "pos_z": -99.09375,
+ "stations": "Gabriel's Sanctuary 23408"
+ },
+ {
+ "name": "Nata",
+ "pos_x": 137.46875,
+ "pos_y": -186.96875,
+ "pos_z": 41.75,
+ "stations": "Bowen Keep,Sabine Landing,Wallerstein Survey"
+ },
+ {
+ "name": "Natar",
+ "pos_x": 77.3125,
+ "pos_y": -133.53125,
+ "pos_z": 154.90625,
+ "stations": "Heinkel's Exile,Wickramasinghe Landing"
+ },
+ {
+ "name": "Natay",
+ "pos_x": -53.34375,
+ "pos_y": -158.78125,
+ "pos_z": -18.15625,
+ "stations": "H. G. Wells Sanctuary,Bova Landing"
+ },
+ {
+ "name": "Natera",
+ "pos_x": -37.84375,
+ "pos_y": -111.6875,
+ "pos_z": 49.5,
+ "stations": "Kirchoff Dock,Born Beacon"
+ },
+ {
+ "name": "Natero Kop",
+ "pos_x": -58.875,
+ "pos_y": 36.5625,
+ "pos_z": -132.4375,
+ "stations": "Yu Platform,Carlisle Installation,Zetford Depot"
+ },
+ {
+ "name": "Natrians",
+ "pos_x": -106.0625,
+ "pos_y": 39.5,
+ "pos_z": 98.71875,
+ "stations": "Henize City,Bowersox Port,Hammond Port,Litke Settlement"
+ },
+ {
+ "name": "Natrimi",
+ "pos_x": 38.28125,
+ "pos_y": -81.46875,
+ "pos_z": 77.96875,
+ "stations": "Tasman Enterprise,Biesbroeck Horizons,Struve Installation"
+ },
+ {
+ "name": "Nauacan",
+ "pos_x": 22.25,
+ "pos_y": -101.03125,
+ "pos_z": -56.25,
+ "stations": "Precourt Port"
+ },
+ {
+ "name": "Nauahtunu",
+ "pos_x": 169.15625,
+ "pos_y": 3.28125,
+ "pos_z": 55.125,
+ "stations": "Brunner Survey,Shiras Terminal,Rodrigues' Folly"
+ },
+ {
+ "name": "Naualam",
+ "pos_x": 113.71875,
+ "pos_y": -56.4375,
+ "pos_z": 4.3125,
+ "stations": "Galois Settlement,Pogson City,Gould Vision,Stone Estate,Davidson Silo"
+ },
+ {
+ "name": "Nauan Du",
+ "pos_x": -30.375,
+ "pos_y": 156.4375,
+ "pos_z": -12.4375,
+ "stations": "Danvers Depot,Crumey Platform,Herndon Escape"
+ },
+ {
+ "name": "Nauana",
+ "pos_x": 77.15625,
+ "pos_y": -94.09375,
+ "pos_z": 96.65625,
+ "stations": "Bowen Terminal,May Forum,Oshima Hub"
+ },
+ {
+ "name": "Nauani",
+ "pos_x": 81.375,
+ "pos_y": -51.1875,
+ "pos_z": -14,
+ "stations": "Lanchester's Folly,Vaucanson Station,Thomson Orbital,Morey Enterprise,Ackerman Vista"
+ },
+ {
+ "name": "Nauksas",
+ "pos_x": -86.75,
+ "pos_y": 40.84375,
+ "pos_z": 119.09375,
+ "stations": "Markov's Progress,Tull Prospect"
+ },
+ {
+ "name": "Naunapitana",
+ "pos_x": 119.59375,
+ "pos_y": -178.28125,
+ "pos_z": 2.71875,
+ "stations": "Chadbourne Works"
+ },
+ {
+ "name": "Naunei",
+ "pos_x": 100.75,
+ "pos_y": -62.625,
+ "pos_z": 10.75,
+ "stations": "Cuffey Orbital,Al-Khujandi Plant,Boole Installation,Kelly Dock,Kelly Orbital,Gill Port,Merril Refinery,Ingstad Arena,Mooz Port,Common Port,Flynn Hub,Doctorow Prospect,Tousey Horizons"
+ },
+ {
+ "name": "Naunet",
+ "pos_x": -9.40625,
+ "pos_y": -20.15625,
+ "pos_z": -155.40625,
+ "stations": "Zelazny Gateway,McKee Base,Wallace Gateway,Steakley Mines,Akers Mines"
+ },
+ {
+ "name": "Naungni",
+ "pos_x": 184.375,
+ "pos_y": -173.1875,
+ "pos_z": -15.3125,
+ "stations": "Harvia Landing,Wilson Oasis"
+ },
+ {
+ "name": "Nauni",
+ "pos_x": -33.5,
+ "pos_y": -70.1875,
+ "pos_z": -162.1875,
+ "stations": "Beaufoy Vision,Rothman Terminal,Rescue Ship - Beaufoy Vision"
+ },
+ {
+ "name": "Naunin",
+ "pos_x": 55.34375,
+ "pos_y": -13.1875,
+ "pos_z": 76.125,
+ "stations": "Diamond Station,Zamyatin Station,MacLeod Hub,Asimov Gateway,Crown Survey"
+ },
+ {
+ "name": "Nauo",
+ "pos_x": -46.40625,
+ "pos_y": 7.03125,
+ "pos_z": 122.09375,
+ "stations": "Cook Reach"
+ },
+ {
+ "name": "Nauolung",
+ "pos_x": 133.6875,
+ "pos_y": -159.8125,
+ "pos_z": -37.28125,
+ "stations": "Halley Orbital,Hornoch City,Scheerbart Barracks"
+ },
+ {
+ "name": "Naura Mol",
+ "pos_x": 70.3125,
+ "pos_y": -195.96875,
+ "pos_z": -30.78125,
+ "stations": "Petlyakov Vision,Shaw Beacon,Kagawa Survey,Cantor Terminal"
+ },
+ {
+ "name": "Nauracota",
+ "pos_x": -44.71875,
+ "pos_y": -9.75,
+ "pos_z": 170,
+ "stations": "Silves Hangar,Hughes Terminal,Grimwood Reach"
+ },
+ {
+ "name": "Nauragwa",
+ "pos_x": 103.59375,
+ "pos_y": 78.25,
+ "pos_z": -68.65625,
+ "stations": "Nelson Platform,Xing Settlement"
+ },
+ {
+ "name": "Nauravanec",
+ "pos_x": 106.6875,
+ "pos_y": 120.875,
+ "pos_z": -3.53125,
+ "stations": "Wandrei Base,Scortia Port,Noli Base"
+ },
+ {
+ "name": "Nauresanoy",
+ "pos_x": 146.84375,
+ "pos_y": -180,
+ "pos_z": 11,
+ "stations": "Emshwiller's Claim"
+ },
+ {
+ "name": "Nauri",
+ "pos_x": 107.96875,
+ "pos_y": -32.96875,
+ "pos_z": 35.875,
+ "stations": "Hiraga Dock"
+ },
+ {
+ "name": "Naurung",
+ "pos_x": -48.65625,
+ "pos_y": -42,
+ "pos_z": -139.34375,
+ "stations": "Frick Base"
+ },
+ {
+ "name": "Navajo",
+ "pos_x": -34.0625,
+ "pos_y": 23.53125,
+ "pos_z": 130.78125,
+ "stations": "Akers Depot,Elgin Beacon"
+ },
+ {
+ "name": "Navajoar",
+ "pos_x": -69.59375,
+ "pos_y": -18.46875,
+ "pos_z": 67.125,
+ "stations": "Denton Observatory,Dayuan Platform"
+ },
+ {
+ "name": "Navajojiu",
+ "pos_x": -48.9375,
+ "pos_y": 46.46875,
+ "pos_z": 120.0625,
+ "stations": "Deluc Survey"
+ },
+ {
+ "name": "Navas",
+ "pos_x": 155.4375,
+ "pos_y": 10.59375,
+ "pos_z": 25.3125,
+ "stations": "Jones Landing,Neumann Colony,Coney Hub"
+ },
+ {
+ "name": "Nawa",
+ "pos_x": 138.6875,
+ "pos_y": -76.03125,
+ "pos_z": 34.21875,
+ "stations": "Quetelet Dock,Rosseland Station,Fedden Terminal,Loewy Station,Derleth's Progress"
+ },
+ {
+ "name": "Nawad",
+ "pos_x": -81.90625,
+ "pos_y": 20.15625,
+ "pos_z": -81.5,
+ "stations": "Clifford Station,Muhammad Ibn Battuta Bastion,Boming Dock"
+ },
+ {
+ "name": "Nawait",
+ "pos_x": 143.84375,
+ "pos_y": -80.59375,
+ "pos_z": -56.875,
+ "stations": "Narlikar Station,Phillips Terminal,Ahnert-Rohlfs Terminal,Puleston Landing,Kurland Lab"
+ },
+ {
+ "name": "Nawer",
+ "pos_x": -25.375,
+ "pos_y": 32.5625,
+ "pos_z": 154.65625,
+ "stations": "Menezes Arena,Yolen Reformatory"
+ },
+ {
+ "name": "Naxixo",
+ "pos_x": -57.4375,
+ "pos_y": -139,
+ "pos_z": 23.28125,
+ "stations": "Hanke-Woods Platform,Blaauw Dock,al-Kashi Station,Carpenter Landing,Veblen Installation"
+ },
+ {
+ "name": "Nayahun",
+ "pos_x": -122.53125,
+ "pos_y": 28.4375,
+ "pos_z": 28.65625,
+ "stations": "Hirase Dock,Chapman Gateway,Hopkins Port,Sharman Dock,Wilson Terminal,McCoy Terminal"
+ },
+ {
+ "name": "Nayanezgani",
+ "pos_x": 51.34375,
+ "pos_y": -177.03125,
+ "pos_z": 15.96875,
+ "stations": "Bailly Landing,Compton Arsenal"
+ },
+ {
+ "name": "Nayapota",
+ "pos_x": 45.75,
+ "pos_y": 36.375,
+ "pos_z": -82.8125,
+ "stations": "Aguirre Survey,Francisco de Eliza Mine"
+ },
+ {
+ "name": "Ndaakoshi",
+ "pos_x": 77.5625,
+ "pos_y": -124.15625,
+ "pos_z": -75.125,
+ "stations": "Paul Hangar,Hussenot Dock,Alpers Survey"
+ },
+ {
+ "name": "Ndaba",
+ "pos_x": 11.53125,
+ "pos_y": -141.84375,
+ "pos_z": -134.90625,
+ "stations": "Khrenov Terminal,Vlamingh Survey"
+ },
+ {
+ "name": "Ndabangluya",
+ "pos_x": 2.84375,
+ "pos_y": 17.40625,
+ "pos_z": -103.21875,
+ "stations": "Melvin City"
+ },
+ {
+ "name": "Ndabien",
+ "pos_x": 46.40625,
+ "pos_y": -48.125,
+ "pos_z": -123.1875,
+ "stations": "Konscak Enterprise,Lenoir Depot,Macan Station"
+ },
+ {
+ "name": "Ndan",
+ "pos_x": 136.5,
+ "pos_y": -20.34375,
+ "pos_z": 32.875,
+ "stations": "Lippisch Point,Goldberg Survey"
+ },
+ {
+ "name": "Ndanbain",
+ "pos_x": 41.5625,
+ "pos_y": 139.6875,
+ "pos_z": -20.34375,
+ "stations": "Linteris Orbital,Gregory Enterprise,Lambert Enterprise"
+ },
+ {
+ "name": "Ndandjimbo",
+ "pos_x": -98,
+ "pos_y": -45.375,
+ "pos_z": 44.5625,
+ "stations": "Brunel Hangar,Grunsfeld Plant"
+ },
+ {
+ "name": "Ndano",
+ "pos_x": 173.84375,
+ "pos_y": -213.21875,
+ "pos_z": -36.25,
+ "stations": "Goryu Terminal,Severin Settlement"
+ },
+ {
+ "name": "Ndebech",
+ "pos_x": 59.53125,
+ "pos_y": -100.375,
+ "pos_z": -23.5,
+ "stations": "Lemonnier Colony,Leydenfrost Base,Bereznyak Installation"
+ },
+ {
+ "name": "Ndeber",
+ "pos_x": -66.78125,
+ "pos_y": -59.84375,
+ "pos_z": 83.53125,
+ "stations": "Onufriyenko Mine,Tryggvason Escape"
+ },
+ {
+ "name": "Nden",
+ "pos_x": -17.4375,
+ "pos_y": -0.84375,
+ "pos_z": 139.3125,
+ "stations": "Edison Beacon,de Balboa Mines,Rontgen Outpost,Qureshi Enterprise"
+ },
+ {
+ "name": "Ndenaiwae",
+ "pos_x": -34.125,
+ "pos_y": -158.625,
+ "pos_z": -68.8125,
+ "stations": "Bethke Colony,Spielberg Vision,Liouville Refinery"
+ },
+ {
+ "name": "Ndjabog",
+ "pos_x": -88.3125,
+ "pos_y": -88.21875,
+ "pos_z": -44.84375,
+ "stations": "Malenchenko Port,Crown Holdings,Burbank Gateway,Armstrong Installation,Napier Beacon"
+ },
+ {
+ "name": "Ndjala",
+ "pos_x": 19.6875,
+ "pos_y": -156.4375,
+ "pos_z": 139.5625,
+ "stations": "Sitter Port"
+ },
+ {
+ "name": "Ndjari",
+ "pos_x": -137.59375,
+ "pos_y": 36,
+ "pos_z": 2.09375,
+ "stations": "Kidman Terminal,Foucault Dock,Burnell Terminal,Williams' Folly,Roberts Holdings"
+ },
+ {
+ "name": "Ndoyenghus",
+ "pos_x": -31.28125,
+ "pos_y": -127.03125,
+ "pos_z": 28.5625,
+ "stations": "Whitford Base,Nilson Port,Binney Ring,Lewis Enterprise"
+ },
+ {
+ "name": "Ndoyerunga",
+ "pos_x": 108,
+ "pos_y": -54.625,
+ "pos_z": -93.1875,
+ "stations": "Kirtley Horizons,Kelly Base,Zettel Installation,Cole Gateway,Wells Colony"
+ },
+ {
+ "name": "Ndozins",
+ "pos_x": -14.3125,
+ "pos_y": -10.6875,
+ "pos_z": -60.5625,
+ "stations": "Coney Arena"
+ },
+ {
+ "name": "Ndria",
+ "pos_x": -56.8125,
+ "pos_y": 31.9375,
+ "pos_z": -87.375,
+ "stations": "McMonagle Enterprise,Nicolet Horizons,Sagan Gateway,Crichton Landing,Disch Keep"
+ },
+ {
+ "name": "Ndriamente",
+ "pos_x": -56.1875,
+ "pos_y": -5.5,
+ "pos_z": 164.21875,
+ "stations": "Guidoni Port,Blenkinsop Platform"
+ },
+ {
+ "name": "Ndrian",
+ "pos_x": 56.65625,
+ "pos_y": 106.90625,
+ "pos_z": -104.53125,
+ "stations": "Bulleid Settlement,Lind Station,Grimwood Survey,Steinmuller Landing,Arrhenius City"
+ },
+ {
+ "name": "Neali",
+ "pos_x": 85.03125,
+ "pos_y": -26.4375,
+ "pos_z": -14.40625,
+ "stations": "Melnick Survey,Bohnhoff's Exile,Butz Platform"
+ },
+ {
+ "name": "Neb Djen",
+ "pos_x": 42.65625,
+ "pos_y": 131.5625,
+ "pos_z": -79.71875,
+ "stations": "Lindbohm Survey,Lasswitz Relay"
+ },
+ {
+ "name": "Neb Huldr",
+ "pos_x": 102.4375,
+ "pos_y": -73.4375,
+ "pos_z": 56.4375,
+ "stations": "Dall Mines,Keldysh Terminal,Piano Holdings"
+ },
+ {
+ "name": "Nebtete",
+ "pos_x": 59.28125,
+ "pos_y": 44.375,
+ "pos_z": 16.6875,
+ "stations": "Bagian Vision,Korzun Gateway,Hume Landing"
+ },
+ {
+ "name": "Nebther",
+ "pos_x": 135.96875,
+ "pos_y": 37.15625,
+ "pos_z": -3.59375,
+ "stations": "Salgari Dock"
+ },
+ {
+ "name": "Nebthet",
+ "pos_x": 4,
+ "pos_y": -9.96875,
+ "pos_z": -128.46875,
+ "stations": "Garneau Port,Frick Landing,Satcher Horizons,Gernsback Refinery"
+ },
+ {
+ "name": "Nech Kairu",
+ "pos_x": -31.78125,
+ "pos_y": 100.03125,
+ "pos_z": 29.65625,
+ "stations": "Anthony Terminal,Tanaka Landing,Atiyah Mine"
+ },
+ {
+ "name": "Neche",
+ "pos_x": 63.6875,
+ "pos_y": 12.90625,
+ "pos_z": -13.09375,
+ "stations": "Descartes Station,Kozeyev Beacon,Leeuwenhoek Station,Tem Bastion"
+ },
+ {
+ "name": "Ned Erh",
+ "pos_x": 6.34375,
+ "pos_y": -111.65625,
+ "pos_z": 0.4375,
+ "stations": "Polyakov Hangar,Brunner Refinery,Carver Hub,Springer Lab"
+ },
+ {
+ "name": "Nedos",
+ "pos_x": -72.71875,
+ "pos_y": -43.21875,
+ "pos_z": -24.40625,
+ "stations": "Readdy City"
+ },
+ {
+ "name": "Nefen",
+ "pos_x": 1.59375,
+ "pos_y": -23,
+ "pos_z": 117.15625,
+ "stations": "Prunariu Port,Wiberg Penal colony,Morgan Landing"
+ },
+ {
+ "name": "Nefes",
+ "pos_x": -59.46875,
+ "pos_y": -146.40625,
+ "pos_z": 6.34375,
+ "stations": "Kovalevsky Base,Bluford Orbital,Banach Terminal"
+ },
+ {
+ "name": "Nega",
+ "pos_x": -93.0625,
+ "pos_y": 23.59375,
+ "pos_z": 150.0625,
+ "stations": "Linaweaver Beacon"
+ },
+ {
+ "name": "Negafook",
+ "pos_x": -49.21875,
+ "pos_y": 9.46875,
+ "pos_z": -155.65625,
+ "stations": "Fernandes Enterprise,Cardano Vision,MacVicar's Folly"
+ },
+ {
+ "name": "Neganhot",
+ "pos_x": 77.78125,
+ "pos_y": 50.9375,
+ "pos_z": 79.96875,
+ "stations": "He Landing,Pournelle Silo,Gardner Settlement"
+ },
+ {
+ "name": "Negani",
+ "pos_x": 70.65625,
+ "pos_y": -53.875,
+ "pos_z": -22.25,
+ "stations": "Thiele Point,Einstein Mines"
+ },
+ {
+ "name": "Negas",
+ "pos_x": 164.25,
+ "pos_y": -183.625,
+ "pos_z": -21.09375,
+ "stations": "Kranz Prospect"
+ },
+ {
+ "name": "Negasargun",
+ "pos_x": 6.125,
+ "pos_y": -74.15625,
+ "pos_z": 84.40625,
+ "stations": "Murray Ring"
+ },
+ {
+ "name": "Negasta",
+ "pos_x": -21.4375,
+ "pos_y": 139.875,
+ "pos_z": 21.5625,
+ "stations": "Farmer Dock,Carr Dock,Ballard Dock"
+ },
+ {
+ "name": "Negi",
+ "pos_x": -31.21875,
+ "pos_y": 21,
+ "pos_z": -139.40625,
+ "stations": "Cortes Horizons,Rose Refinery"
+ },
+ {
+ "name": "Negidals",
+ "pos_x": -63.8125,
+ "pos_y": -28.25,
+ "pos_z": 76.1875,
+ "stations": "Neumann Market,Perry Dock,Vonarburg Station,Bruce Terminal,Bayley Dock,Dalmas Hub,Crown Palace,Tolstoi Plant,Schade Port,Tsibliyev Installation"
+ },
+ {
+ "name": "Negin",
+ "pos_x": -2.625,
+ "pos_y": 153.875,
+ "pos_z": -28.25,
+ "stations": "Hahn Orbital,Peral Gateway,Gentle Terminal,Morgan Gateway,Carlisle Settlement,Mawson Landing"
+ },
+ {
+ "name": "Neginn",
+ "pos_x": 19.28125,
+ "pos_y": -202.875,
+ "pos_z": -40.8125,
+ "stations": "Delporte Vision"
+ },
+ {
+ "name": "Negir",
+ "pos_x": 154.5625,
+ "pos_y": -187.53125,
+ "pos_z": -4.125,
+ "stations": "Theodor Winnecke Base,Babcock's Inheritance,Hughes' Progress"
+ },
+ {
+ "name": "Negri",
+ "pos_x": 188.5,
+ "pos_y": -173,
+ "pos_z": 78.875,
+ "stations": "Sukhoi Terminal,Johri Port,Ilyushin Dock,McMahon Lab,Celebi Installation"
+ },
+ {
+ "name": "Negrici",
+ "pos_x": 188.78125,
+ "pos_y": -158.125,
+ "pos_z": 41,
+ "stations": "Al Sufi Port,Martin Survey"
+ },
+ {
+ "name": "Negriti",
+ "pos_x": -36.65625,
+ "pos_y": 40.34375,
+ "pos_z": -69.03125,
+ "stations": "Neumann Survey,Thompson Vision,Herjulfsson Refinery"
+ },
+ {
+ "name": "Negrito",
+ "pos_x": 78.40625,
+ "pos_y": -82.59375,
+ "pos_z": 10.90625,
+ "stations": "Rolland Station,Steakley Station,Walker Dock,Hynek Landing,Shatner Works,Hill Works,Friend Prospect,Abe City,Barnwell Enterprise,Gaspar de Lemos Enterprise,Salgari Hub"
+ },
+ {
+ "name": "Nehabtani",
+ "pos_x": 21.34375,
+ "pos_y": -247.375,
+ "pos_z": 77.03125,
+ "stations": "Russell Terminal,Ziegel Orbital"
+ },
+ {
+ "name": "Nehebayo",
+ "pos_x": -70.28125,
+ "pos_y": -76.6875,
+ "pos_z": 46,
+ "stations": "George's Progress,Manning Survey,Rothman Platform"
+ },
+ {
+ "name": "Nehebkau",
+ "pos_x": -16.6875,
+ "pos_y": -120.75,
+ "pos_z": -30.40625,
+ "stations": "Herbert Platform"
+ },
+ {
+ "name": "Nehet",
+ "pos_x": 81.5,
+ "pos_y": -116.46875,
+ "pos_z": 37.03125,
+ "stations": "Xuesen Orbital,Tomita Port,Tennyson d'Eyncourt Silo,Delaunay Port"
+ },
+ {
+ "name": "Neites",
+ "pos_x": 156.21875,
+ "pos_y": -88.53125,
+ "pos_z": -37.09375,
+ "stations": "Giger Dock,Mattei Landing"
+ },
+ {
+ "name": "Neits",
+ "pos_x": 9.84375,
+ "pos_y": -68.28125,
+ "pos_z": -9.875,
+ "stations": "Still Hangar,Leckie Prospect,Watson Settlement,Crown Port"
+ },
+ {
+ "name": "Neitsi",
+ "pos_x": -134.25,
+ "pos_y": -99.9375,
+ "pos_z": 42.9375,
+ "stations": "Scully-Power Base"
+ },
+ {
+ "name": "Nekhbet",
+ "pos_x": -15.375,
+ "pos_y": -26.78125,
+ "pos_z": -59.78125,
+ "stations": "Joliot-Curie Hangar,Broglie Point,Sacco Plant"
+ },
+ {
+ "name": "Nekhmetona",
+ "pos_x": 91.09375,
+ "pos_y": -32.125,
+ "pos_z": -107.375,
+ "stations": "MacLean Stop"
+ },
+ {
+ "name": "Nemaiamburi",
+ "pos_x": -8.46875,
+ "pos_y": -22.46875,
+ "pos_z": 103.59375,
+ "stations": "Krusvar Relay,Ogden Prospect,Boswell's Folly,Morris Port"
+ },
+ {
+ "name": "Nemasty",
+ "pos_x": -86.09375,
+ "pos_y": -130.3125,
+ "pos_z": 57.28125,
+ "stations": "Napier Orbital,Stevin Terminal,Peano Camp"
+ },
+ {
+ "name": "Nemedet",
+ "pos_x": 19.90625,
+ "pos_y": -119.71875,
+ "pos_z": 99.65625,
+ "stations": "Tanaka Bastion,Vuia Colony"
+ },
+ {
+ "name": "Nemehi",
+ "pos_x": 19.40625,
+ "pos_y": -9.96875,
+ "pos_z": 39.46875,
+ "stations": "Gordon Depot,Haipeng Orbital,Stephenson Orbital,Meikle Port,Kaku Dock,Shepherd Hub,Hutton Port,Ocampo City,al-Khowarizmi Hub,Laird Lab,Wheelock Base,Furukawa Terminal,Leclerc Hub,Kuipers Orbital"
+ },
+ {
+ "name": "Nemehim",
+ "pos_x": 141.0625,
+ "pos_y": 49.5,
+ "pos_z": 49.21875,
+ "stations": "Poleshchuk Dock,Avdeyev Terminal"
+ },
+ {
+ "name": "Nemehutas",
+ "pos_x": 146.3125,
+ "pos_y": 71.21875,
+ "pos_z": -83.3125,
+ "stations": "Ozanne Platform"
+ },
+ {
+ "name": "Nemeno",
+ "pos_x": -75.4375,
+ "pos_y": -133.75,
+ "pos_z": 92.84375,
+ "stations": "Reightler Works,Siemens Terminal,Akiyama Dock"
+ },
+ {
+ "name": "Nemepawe",
+ "pos_x": -127.59375,
+ "pos_y": 34.90625,
+ "pos_z": -43.40625,
+ "stations": "Mitchell Port"
+ },
+ {
+ "name": "Nemet",
+ "pos_x": 10.0625,
+ "pos_y": -61.09375,
+ "pos_z": 17.25,
+ "stations": "Hirase Horizons,Garan Dock,Prunariu Prospect,Gwynn Keep"
+ },
+ {
+ "name": "Nemetan",
+ "pos_x": -50.5,
+ "pos_y": 18.25,
+ "pos_z": 146.3125,
+ "stations": "Mitchell Dock,Fernao do Po Terminal,Dummer Survey,Clairaut Beacon"
+ },
+ {
+ "name": "Nemetati",
+ "pos_x": 52.09375,
+ "pos_y": -158.0625,
+ "pos_z": 135.75,
+ "stations": "Hogg Platform,Duque Horizons,Friend Penal colony"
+ },
+ {
+ "name": "Nemetes",
+ "pos_x": -112.1875,
+ "pos_y": 74.40625,
+ "pos_z": 28.46875,
+ "stations": "Cabral Port,Pavlou Hub,Young Port,Wul Barracks"
+ },
+ {
+ "name": "Nemetu",
+ "pos_x": -44.875,
+ "pos_y": 103.375,
+ "pos_z": -116.09375,
+ "stations": "Asaro Gateway,Younghusband Gateway,Reynolds Vision,Carpini Horizons"
+ },
+ {
+ "name": "Nemgla",
+ "pos_x": 119.65625,
+ "pos_y": -96.5,
+ "pos_z": -12.15625,
+ "stations": "Maine's Inheritance,Norman Point"
+ },
+ {
+ "name": "Nenexo",
+ "pos_x": -150.1875,
+ "pos_y": -50.21875,
+ "pos_z": -3.5625,
+ "stations": "Ciferri Ring,Hilmers Horizons,Clement Prospect,Peano Vista"
+ },
+ {
+ "name": "Nepehuevit",
+ "pos_x": 137.8125,
+ "pos_y": -123.71875,
+ "pos_z": 66.21875,
+ "stations": "Lynden-Bell Orbital,Malcolm Beacon,Sato Orbital"
+ },
+ {
+ "name": "Neperimpox",
+ "pos_x": 72.71875,
+ "pos_y": 91.09375,
+ "pos_z": 125.75,
+ "stations": "Rothfuss Mines"
+ },
+ {
+ "name": "Nepi",
+ "pos_x": 37.4375,
+ "pos_y": -20.8125,
+ "pos_z": 58.84375,
+ "stations": "Ron Hubbard Vision,Carrasco Orbital,Vinge Stop,Bosch Refinery,Maire Gateway,Cayley's Folly,Hovgaard's Claim,Pohl's Folly,Thoreau Installation"
+ },
+ {
+ "name": "Neris",
+ "pos_x": -111.625,
+ "pos_y": -109.125,
+ "pos_z": -55.71875,
+ "stations": "Wafer Dock,Godel Co-operative,Mohun Station,Popov Holdings"
+ },
+ {
+ "name": "Nerishis",
+ "pos_x": 106.78125,
+ "pos_y": -32.34375,
+ "pos_z": -53.1875,
+ "stations": "Nespoli Port"
+ },
+ {
+ "name": "Neritus",
+ "pos_x": 75.4375,
+ "pos_y": 2.625,
+ "pos_z": -30.875,
+ "stations": "Toll Ring,Egan Silo,Lambert Lab,Weinbaum Arsenal,Leichhardt Station"
+ },
+ {
+ "name": "Nerre",
+ "pos_x": 80.03125,
+ "pos_y": 15.75,
+ "pos_z": 139.75,
+ "stations": "Felice Platform,Grijalva Orbital,Corte-Real Hub,Coles Installation"
+ },
+ {
+ "name": "Nerremaia",
+ "pos_x": 169.875,
+ "pos_y": -200.375,
+ "pos_z": 71.09375,
+ "stations": "Barcelona Port,Marcy Relay"
+ },
+ {
+ "name": "Nerrete",
+ "pos_x": 185.78125,
+ "pos_y": -127.1875,
+ "pos_z": 4.9375,
+ "stations": "McMillan Landing,Danjon Base"
+ },
+ {
+ "name": "Nerry",
+ "pos_x": -125.96875,
+ "pos_y": -17.46875,
+ "pos_z": 80.46875,
+ "stations": "Speke Enterprise,Cady Town,Brongniart's Progress"
+ },
+ {
+ "name": "Nerthus",
+ "pos_x": 15.5625,
+ "pos_y": -57.96875,
+ "pos_z": 37.75,
+ "stations": "Muller Station,Tago Dock,Extra Vision,Shirley Relay"
+ },
+ {
+ "name": "Nervi",
+ "pos_x": -74.75,
+ "pos_y": -72.71875,
+ "pos_z": 35.84375,
+ "stations": "Savitskaya Vision,Al-Kashi Terminal,Davis Arsenal"
+ },
+ {
+ "name": "Nervii",
+ "pos_x": 114.5625,
+ "pos_y": 44.65625,
+ "pos_z": -107.78125,
+ "stations": "Grzimek Relay,Qian's Pride"
+ },
+ {
+ "name": "Nervir",
+ "pos_x": 39.84375,
+ "pos_y": -24.15625,
+ "pos_z": -43.21875,
+ "stations": "Metcalf Dock,Musa al-Khwarizmi Ring,McCoy Terminal,Velho Holdings,Goeppert-Mayer Terminal,Wallis Hub,Sladek Installation,Parry Enterprise,Werner von Siemens City,Burbank Port,Curbeam Terminal"
+ },
+ {
+ "name": "Nerviri",
+ "pos_x": 43.5,
+ "pos_y": -150.9375,
+ "pos_z": -55.28125,
+ "stations": "Galle's Exile"
+ },
+ {
+ "name": "Nespel",
+ "pos_x": 25.96875,
+ "pos_y": -171.09375,
+ "pos_z": -5.40625,
+ "stations": "Burnham City,Merritt Port"
+ },
+ {
+ "name": "Nespeleve",
+ "pos_x": 68,
+ "pos_y": -141.28125,
+ "pos_z": -105.25,
+ "stations": "Houtman Vision,Wilkes Orbital,Huxley Dock,Woolley Base,Acharya Station,Westerhout Penal colony"
+ },
+ {
+ "name": "Nespeli",
+ "pos_x": 141.1875,
+ "pos_y": 42.59375,
+ "pos_z": 35.15625,
+ "stations": "Leclerc Gateway,Poleshchuk Hub,Furukawa Enterprise"
+ },
+ {
+ "name": "Nespell",
+ "pos_x": 158.5,
+ "pos_y": -96.96875,
+ "pos_z": 38,
+ "stations": "Schiaparelli Port,Reid Dock,Dingle Enterprise"
+ },
+ {
+ "name": "Neto",
+ "pos_x": -41.1875,
+ "pos_y": 7.65625,
+ "pos_z": 36.3125,
+ "stations": "Ising Vision,Citroen Hub"
+ },
+ {
+ "name": "Nevermore",
+ "pos_x": 19.375,
+ "pos_y": 88.28125,
+ "pos_z": -10.15625,
+ "stations": "Pinto Ring,Borel Enterprise"
+ },
+ {
+ "name": "New Yembo",
+ "pos_x": -334.625,
+ "pos_y": -4.46875,
+ "pos_z": 73.5,
+ "stations": "Unity,VanderMeer Landing,Tevis Terminal"
+ },
+ {
+ "name": "Nez Pelliri",
+ "pos_x": -55.125,
+ "pos_y": -31.59375,
+ "pos_z": 58.375,
+ "stations": "Jordan Landing"
+ },
+ {
+ "name": "Nez Perce",
+ "pos_x": -78.53125,
+ "pos_y": -21.3125,
+ "pos_z": -58.8125,
+ "stations": "Tsibliyev Installation,Afanasyev Holdings,Turtledove Penal colony"
+ },
+ {
+ "name": "Nezhen",
+ "pos_x": 9.5,
+ "pos_y": -105.9375,
+ "pos_z": 120.96875,
+ "stations": "Hand Dock,Mallett Relay"
+ },
+ {
+ "name": "Nezheng",
+ "pos_x": -100.53125,
+ "pos_y": 23.65625,
+ "pos_z": 141.1875,
+ "stations": "Viehbock Relay"
+ },
+ {
+ "name": "Nezhenses",
+ "pos_x": 6.25,
+ "pos_y": -41,
+ "pos_z": -27.03125,
+ "stations": "Babbage Hub"
+ },
+ {
+ "name": "Ngadakhis",
+ "pos_x": 34.6875,
+ "pos_y": -45.53125,
+ "pos_z": 76.625,
+ "stations": "Murray Hub,Copernicus Orbital,Shirley Settlement,Detmer Hub,Cugnot Base"
+ },
+ {
+ "name": "Ngadandari",
+ "pos_x": 62.75,
+ "pos_y": -74.1875,
+ "pos_z": 109.5,
+ "stations": "Whitelaw Mines,Napier Terminal,Pellegrino Landing"
+ },
+ {
+ "name": "Ngadjal",
+ "pos_x": 67.75,
+ "pos_y": -109.75,
+ "pos_z": 51.59375,
+ "stations": "Lundmark Prospect,Gotz Platform,Lundwall Relay"
+ },
+ {
+ "name": "Ngadji",
+ "pos_x": 55.71875,
+ "pos_y": -196.53125,
+ "pos_z": -33.375,
+ "stations": "Youll Mines,Youll Hub,Beriev Works"
+ },
+ {
+ "name": "Ngadjuk",
+ "pos_x": 150.40625,
+ "pos_y": -95.5625,
+ "pos_z": 113.78125,
+ "stations": "Becvar Enterprise,Schoening Dock,Flaugergues Station,Lupoff Vision,Baxter Vista"
+ },
+ {
+ "name": "Ngaduni",
+ "pos_x": 46.65625,
+ "pos_y": -93.40625,
+ "pos_z": 91.53125,
+ "stations": "Hind Landing"
+ },
+ {
+ "name": "Ngaiawang",
+ "pos_x": 62.375,
+ "pos_y": -89.90625,
+ "pos_z": -13,
+ "stations": "de Sousa Beacon,Emshwiller City,Dornier Terminal,MacGill Orbital,Chern Barracks"
+ },
+ {
+ "name": "Ngaislan",
+ "pos_x": -128.40625,
+ "pos_y": 33.59375,
+ "pos_z": -24.75,
+ "stations": "Swigert Terminal,Vinogradov Port,Ising Colony,Hawke Depot,Fuca Works"
+ },
+ {
+ "name": "Ngaits",
+ "pos_x": 19.5625,
+ "pos_y": 78.28125,
+ "pos_z": -140.09375,
+ "stations": "Otiman Mines,Buchli Base"
+ },
+ {
+ "name": "Ngakwakan",
+ "pos_x": -126.5625,
+ "pos_y": -78.53125,
+ "pos_z": 52.9375,
+ "stations": "Pashin Dock"
+ },
+ {
+ "name": "Ngakwilo",
+ "pos_x": -42.46875,
+ "pos_y": 187.5625,
+ "pos_z": -26.34375,
+ "stations": "Friend Landing,Iqbal Depot"
+ },
+ {
+ "name": "Ngakwites",
+ "pos_x": 6.8125,
+ "pos_y": -161.5,
+ "pos_z": 73.65625,
+ "stations": "Seyfert Port,Izumikawa City,Barmin Ring,Melotte Penal colony,Houtman Observatory,d'Eyncourt Beacon"
+ },
+ {
+ "name": "Ngalakan",
+ "pos_x": 154.25,
+ "pos_y": -176.34375,
+ "pos_z": 97.625,
+ "stations": "Karman Landing,Gaensler Point,Greene Prospect"
+ },
+ {
+ "name": "Ngale",
+ "pos_x": 102.09375,
+ "pos_y": -143.71875,
+ "pos_z": 124.46875,
+ "stations": "Roe Hub,Gill Colony,Elmore Reach"
+ },
+ {
+ "name": "Ngalea",
+ "pos_x": -81.1875,
+ "pos_y": 41.53125,
+ "pos_z": 141.625,
+ "stations": "Cook Penal colony"
+ },
+ {
+ "name": "Ngaledi",
+ "pos_x": -5.65625,
+ "pos_y": -33.71875,
+ "pos_z": 68.375,
+ "stations": "Smith Enterprise,Kavandi City,Springer Terminal,Cayley Orbital,Wankel Port,Smeaton Keep,Coke Port,Burgess' Inheritance,Chalker Vista,Stokes Enterprise"
+ },
+ {
+ "name": "Ngali",
+ "pos_x": 24.3125,
+ "pos_y": -105.84375,
+ "pos_z": 162.78125,
+ "stations": "Chadbourne Settlement,Goodricke Settlement"
+ },
+ {
+ "name": "Ngalia",
+ "pos_x": -74.625,
+ "pos_y": -35.03125,
+ "pos_z": -165.96875,
+ "stations": "Green Enterprise,Kippax Dock,Serling Town,Besonders Works,Tall Enterprise"
+ },
+ {
+ "name": "Ngaliba",
+ "pos_x": -30.5,
+ "pos_y": -8.625,
+ "pos_z": 58.0625,
+ "stations": "Kingsmill Settlement,Nordenskiold Landing"
+ },
+ {
+ "name": "Ngalibamba",
+ "pos_x": 78.71875,
+ "pos_y": -64.28125,
+ "pos_z": 117.53125,
+ "stations": "Edmondson Platform"
+ },
+ {
+ "name": "Ngaliep",
+ "pos_x": 51.28125,
+ "pos_y": -10.34375,
+ "pos_z": 86.09375,
+ "stations": "Moisuc Port,Virtanen Port,Matteucci Enterprise"
+ },
+ {
+ "name": "Ngalinn",
+ "pos_x": 8.625,
+ "pos_y": -219.84375,
+ "pos_z": -60.84375,
+ "stations": "Hickam Survey"
+ },
+ {
+ "name": "Ngalites",
+ "pos_x": 174.03125,
+ "pos_y": -25.625,
+ "pos_z": 36.375,
+ "stations": "Peters Gateway,Tietjen Ring,Arfstrom Survey"
+ },
+ {
+ "name": "Ngalkin",
+ "pos_x": 51.3125,
+ "pos_y": -50.71875,
+ "pos_z": 33.1875,
+ "stations": "Frechet Station,Scalzi Vision,von Bellingshausen Installation,Youll Silo"
+ },
+ {
+ "name": "Ngalu",
+ "pos_x": 35.5,
+ "pos_y": -6.25,
+ "pos_z": 66.3125,
+ "stations": "Redi Enterprise,Polansky Mines"
+ },
+ {
+ "name": "Ngalua",
+ "pos_x": -42.71875,
+ "pos_y": -64.125,
+ "pos_z": 145,
+ "stations": "Haignere Port,Bose Prospect"
+ },
+ {
+ "name": "Ngalung",
+ "pos_x": 92.9375,
+ "pos_y": 40.53125,
+ "pos_z": -33.1875,
+ "stations": "Brackett Port,Wiener Dock,Fujimori Plant"
+ },
+ {
+ "name": "Ngalyod",
+ "pos_x": -50.375,
+ "pos_y": -157.25,
+ "pos_z": 23.71875,
+ "stations": "Cottenot Hub,Tikhonravov Vision,Shen Port,Meyrink Vision,Krigstein Holdings"
+ },
+ {
+ "name": "Ngam",
+ "pos_x": -42.84375,
+ "pos_y": -42.3125,
+ "pos_z": 98.53125,
+ "stations": "Reiter's Folly,Rutherford Terminal,Reightler Terminal,Dyomin Orbital,Bose Orbital,Ferguson Survey,Dowling Base,Tiptree Stop,Bagian Stop,Antonelli Hub"
+ },
+ {
+ "name": "Ngandan",
+ "pos_x": 9.34375,
+ "pos_y": -134.6875,
+ "pos_z": -79.34375,
+ "stations": "Becvar Holdings,Maine Observatory"
+ },
+ {
+ "name": "Ngandavaret",
+ "pos_x": 17.625,
+ "pos_y": 75.09375,
+ "pos_z": -109.40625,
+ "stations": "Dukaj Stop,Alten Settlement"
+ },
+ {
+ "name": "Ngandii",
+ "pos_x": -7.0625,
+ "pos_y": 66.28125,
+ "pos_z": -99.6875,
+ "stations": "Carleson Dock,Remec Survey,von Bellingshausen Gateway,Anvil Survey"
+ },
+ {
+ "name": "Ngandinique",
+ "pos_x": 94.03125,
+ "pos_y": -151.6875,
+ "pos_z": -62.03125,
+ "stations": "Lopez Settlement"
+ },
+ {
+ "name": "Ngandins",
+ "pos_x": 72.09375,
+ "pos_y": 50.8125,
+ "pos_z": 119.90625,
+ "stations": "Cavalieri City,Pinto City,Baffin Horizons,Shipton Depot,Peary Depot"
+ },
+ {
+ "name": "Ngandji",
+ "pos_x": 141.71875,
+ "pos_y": 49.71875,
+ "pos_z": 81.9375,
+ "stations": "Russell Beacon,Hutchinson Camp,Dann Port"
+ },
+ {
+ "name": "Ngandowa",
+ "pos_x": -81.4375,
+ "pos_y": -80.96875,
+ "pos_z": 41.9375,
+ "stations": "Gorbatko Landing,Fisher Orbital,Grandin Hub,Jones Plant"
+ },
+ {
+ "name": "Ngandrusa",
+ "pos_x": 166.5,
+ "pos_y": -104.5625,
+ "pos_z": 100.03125,
+ "stations": "Cherry Terminal"
+ },
+ {
+ "name": "Ngane",
+ "pos_x": 105.5625,
+ "pos_y": -57.03125,
+ "pos_z": 39.59375,
+ "stations": "Oosterhoff Terminal,Parry Camp"
+ },
+ {
+ "name": "Nganeru",
+ "pos_x": 37.625,
+ "pos_y": 35.5,
+ "pos_z": 39.96875,
+ "stations": "Boyle Port,Bykovsky City,Ivanchenkov Orbital,Volta Base"
+ },
+ {
+ "name": "Nganinks",
+ "pos_x": -81.59375,
+ "pos_y": -37.09375,
+ "pos_z": 82.84375,
+ "stations": "Gidzenko Point"
+ },
+ {
+ "name": "Nganjang",
+ "pos_x": 70.25,
+ "pos_y": -5.875,
+ "pos_z": 119.25,
+ "stations": "Braun Orbital,Chamitoff Installation,Hamilton Gateway"
+ },
+ {
+ "name": "Nganji",
+ "pos_x": -24.59375,
+ "pos_y": 36.84375,
+ "pos_z": -132.09375,
+ "stations": "Vizcaino Enterprise,Kanwar's Inheritance,Christian Dock,Jett Settlement,Constantine City,Lanier Dock,Asaro Dock"
+ },
+ {
+ "name": "Ngarabal",
+ "pos_x": -40.625,
+ "pos_y": -135.4375,
+ "pos_z": 7.03125,
+ "stations": "Reber Ring,Dumont Gateway,Jones Vision,Gold's Folly"
+ },
+ {
+ "name": "Ngaradjaras",
+ "pos_x": -91.8125,
+ "pos_y": 62.46875,
+ "pos_z": 147.0625,
+ "stations": "King Terminal,Turzillo Beacon,Rodrigues' Inheritance"
+ },
+ {
+ "name": "Ngarans",
+ "pos_x": -4.9375,
+ "pos_y": -232.96875,
+ "pos_z": 16.40625,
+ "stations": "Tuttle Port,Beshore Landing,Polikarpov Dock,Vinge Beacon"
+ },
+ {
+ "name": "Ngarasini",
+ "pos_x": 188.34375,
+ "pos_y": -112.75,
+ "pos_z": 40.625,
+ "stations": "Barcelona Port,Barmin Settlement,Puiseux Works"
+ },
+ {
+ "name": "Ngarawe",
+ "pos_x": 158.96875,
+ "pos_y": -74.65625,
+ "pos_z": 52.9375,
+ "stations": "Lozino-Lozinskiy Vision,Kreutz Orbital,Metcalf Depot,Adkins Terminal,Linaweaver's Folly"
+ },
+ {
+ "name": "Ngardamayo",
+ "pos_x": 39.53125,
+ "pos_y": 28.53125,
+ "pos_z": -36.21875,
+ "stations": "Buchli City,Weyl Installation,Guidoni Refinery,Otto Landing"
+ },
+ {
+ "name": "Ngardinja",
+ "pos_x": -7.15625,
+ "pos_y": -50.875,
+ "pos_z": 108.25,
+ "stations": "Gaffney Dock,Fleming Gateway,Glashow Ring"
+ },
+ {
+ "name": "Ngarezo",
+ "pos_x": 77.84375,
+ "pos_y": -117.09375,
+ "pos_z": 127.84375,
+ "stations": "Robert Station,Jones Vision"
+ },
+ {
+ "name": "Ngargambo",
+ "pos_x": 15.25,
+ "pos_y": 13.78125,
+ "pos_z": -119.84375,
+ "stations": "Tanaka Landing,Dozois Works"
+ },
+ {
+ "name": "Ngarguna",
+ "pos_x": 26.03125,
+ "pos_y": -21.6875,
+ "pos_z": 96.21875,
+ "stations": "Walheim Dock"
+ },
+ {
+ "name": "Ngari",
+ "pos_x": -55.46875,
+ "pos_y": -8,
+ "pos_z": 142.25,
+ "stations": "Resnick Gateway,McCaffrey Installation,Wilson Survey"
+ },
+ {
+ "name": "Ngarkin",
+ "pos_x": -52.53125,
+ "pos_y": -140.09375,
+ "pos_z": 84.5,
+ "stations": "Berkey Terminal,Bulgarin Point"
+ },
+ {
+ "name": "Ngarkindi",
+ "pos_x": -68.5625,
+ "pos_y": 108.96875,
+ "pos_z": -78.96875,
+ "stations": "Metz Hub,Bates Vision"
+ },
+ {
+ "name": "Ngarokpoi",
+ "pos_x": 51.59375,
+ "pos_y": 42.9375,
+ "pos_z": 57.9375,
+ "stations": "Grzimek Plant"
+ },
+ {
+ "name": "Ngarra",
+ "pos_x": -91.96875,
+ "pos_y": -69.9375,
+ "pos_z": 70.40625,
+ "stations": "Bunch Port,Smith Terminal,Sternberg's Inheritance"
+ },
+ {
+ "name": "Ngarsingo",
+ "pos_x": 146.15625,
+ "pos_y": 41.46875,
+ "pos_z": 13.8125,
+ "stations": "Kerr Freeport,Matheson Dock"
+ },
+ {
+ "name": "Ngartha",
+ "pos_x": -132.25,
+ "pos_y": -78.84375,
+ "pos_z": 24.84375,
+ "stations": "Savinykh Terminal,Carleson's Progress,Ampere Colony,Potrykus Dock"
+ },
+ {
+ "name": "Ngaru",
+ "pos_x": -37.84375,
+ "pos_y": -30.375,
+ "pos_z": 43.46875,
+ "stations": "Shonin Orbital"
+ },
+ {
+ "name": "Ngaruagsa",
+ "pos_x": 68.4375,
+ "pos_y": 14.5,
+ "pos_z": -129.625,
+ "stations": "Yu Refinery,Clarke Station,Rotsler Platform"
+ },
+ {
+ "name": "Ngaruayanka",
+ "pos_x": -23.71875,
+ "pos_y": -32.65625,
+ "pos_z": 28.625,
+ "stations": "Zamka Vision"
+ },
+ {
+ "name": "Ngaruekenas",
+ "pos_x": 42.1875,
+ "pos_y": -10.6875,
+ "pos_z": 62.75,
+ "stations": "Sanger Barracks,Nelson Hub"
+ },
+ {
+ "name": "Ngarut",
+ "pos_x": -130.5625,
+ "pos_y": 68.90625,
+ "pos_z": 99.375,
+ "stations": "Levchenko Landing"
+ },
+ {
+ "name": "Ngath",
+ "pos_x": 114.8125,
+ "pos_y": -34.3125,
+ "pos_z": -44.40625,
+ "stations": "Ocampo's Folly,Piserchia Survey"
+ },
+ {
+ "name": "Ngatha",
+ "pos_x": 126.09375,
+ "pos_y": -36.9375,
+ "pos_z": -59.125,
+ "stations": "Canty Mine,Penzias Arsenal"
+ },
+ {
+ "name": "Ngati",
+ "pos_x": -55.3125,
+ "pos_y": -99.125,
+ "pos_z": -15.03125,
+ "stations": "Fozard Landing,al-Din Point"
+ },
+ {
+ "name": "Ngaun",
+ "pos_x": 165.03125,
+ "pos_y": -139.96875,
+ "pos_z": 101.5,
+ "stations": "Shen Works"
+ },
+ {
+ "name": "Ngauna",
+ "pos_x": -23.90625,
+ "pos_y": 5.1875,
+ "pos_z": -80.65625,
+ "stations": "Young Enterprise"
+ },
+ {
+ "name": "Ngaunetes",
+ "pos_x": 88.84375,
+ "pos_y": -120.84375,
+ "pos_z": 126.59375,
+ "stations": "Ogden Barracks,Anastase Perrotin Depot,Tank Port"
+ },
+ {
+ "name": "Ngauninda",
+ "pos_x": 119.0625,
+ "pos_y": -171.71875,
+ "pos_z": 132.25,
+ "stations": "Rosse Station"
+ },
+ {
+ "name": "Ngauningin",
+ "pos_x": 12.5625,
+ "pos_y": -73.875,
+ "pos_z": 25.0625,
+ "stations": "Bulleid Beacon,Pirsan Landing"
+ },
+ {
+ "name": "Ngawula",
+ "pos_x": 73.625,
+ "pos_y": 56.5625,
+ "pos_z": -39.1875,
+ "stations": "Kerwin Port,Overmyer Survey"
+ },
+ {
+ "name": "Ngbandi",
+ "pos_x": 137.71875,
+ "pos_y": -174.75,
+ "pos_z": 66.25,
+ "stations": "Howe's Pride"
+ },
+ {
+ "name": "Ngbatara",
+ "pos_x": -129.65625,
+ "pos_y": 80.375,
+ "pos_z": -51.28125,
+ "stations": "Malaspina Port"
+ },
+ {
+ "name": "Ngbato",
+ "pos_x": -43.3125,
+ "pos_y": -56.625,
+ "pos_z": 77.84375,
+ "stations": "Stableford Enterprise,Kornbluth Orbital,Hawke City,Gora Hub"
+ },
+ {
+ "name": "Ngbatobii",
+ "pos_x": 13.21875,
+ "pos_y": -38.375,
+ "pos_z": 128,
+ "stations": "Virtanen Prospect,Pinto's Inheritance,Guerrero Gateway,Poncelet Port,Bushkov Orbital,Cartan Point,Asprin Silo"
+ },
+ {
+ "name": "NGC 6188 Sector LC-V c2-28",
+ "pos_x": 1706.1875,
+ "pos_y": -87.625,
+ "pos_z": 4057.15625,
+ "stations": "Morgan's Rock"
+ },
+ {
+ "name": "NGC 7822 Sector BQ-Y d12",
+ "pos_x": -2454.1875,
+ "pos_y": 299.0625,
+ "pos_z": -1326.0625,
+ "stations": "Gorgon Research Facility"
+ },
+ {
+ "name": "Nger",
+ "pos_x": 115.59375,
+ "pos_y": -1.125,
+ "pos_z": -122.28125,
+ "stations": "Walker Port,Farkas Camp,Culpeper Landing"
+ },
+ {
+ "name": "Ngera",
+ "pos_x": -129.40625,
+ "pos_y": -87.1875,
+ "pos_z": 106.59375,
+ "stations": "Ferguson Station,Barnes Orbital,Roggeveen Barracks"
+ },
+ {
+ "name": "Ngeratha",
+ "pos_x": 172.78125,
+ "pos_y": -204.8125,
+ "pos_z": 45.84375,
+ "stations": "Jackson Depot,Lopez Mines"
+ },
+ {
+ "name": "Ngere",
+ "pos_x": 100.78125,
+ "pos_y": -121.78125,
+ "pos_z": -44.78125,
+ "stations": "Babcock Port,d'Allonville Gateway"
+ },
+ {
+ "name": "Ngeri",
+ "pos_x": 33.125,
+ "pos_y": 62.65625,
+ "pos_z": -42.75,
+ "stations": "Kepler Orbital,Sadi Carnot Dock,Samos Installation"
+ },
+ {
+ "name": "Ngerisan",
+ "pos_x": -27.46875,
+ "pos_y": -103.15625,
+ "pos_z": -15.53125,
+ "stations": "Fuca Enterprise,Stuart Enterprise,Verne's Folly"
+ },
+ {
+ "name": "Ngewi",
+ "pos_x": 3.34375,
+ "pos_y": -197.59375,
+ "pos_z": 59,
+ "stations": "Kulin Gateway,Bartini Port,Molchanov Colony"
+ },
+ {
+ "name": "Ngewi'xilak",
+ "pos_x": -52.125,
+ "pos_y": 111,
+ "pos_z": -76.96875,
+ "stations": "Fermat Dock,Paez Station,Gunn Arsenal,Dantec Camp"
+ },
+ {
+ "name": "Ngewin",
+ "pos_x": -115.90625,
+ "pos_y": -122.75,
+ "pos_z": 66.84375,
+ "stations": "Westerfeld Enterprise,Wyndham Settlement"
+ },
+ {
+ "name": "Ngin",
+ "pos_x": 45.25,
+ "pos_y": -0.5,
+ "pos_z": -123.3125,
+ "stations": "Cadamosto Survey,Nordenskiold Depot,Reynolds Beacon"
+ },
+ {
+ "name": "Ngobe",
+ "pos_x": -7.84375,
+ "pos_y": -124.09375,
+ "pos_z": -164.09375,
+ "stations": "Hinz Hub,Arnason Works,Dunyach Point,Koontz Terminal,Ore Orbital,Offutt Colony"
+ },
+ {
+ "name": "Ngobed",
+ "pos_x": 74.3125,
+ "pos_y": -16.53125,
+ "pos_z": -28.375,
+ "stations": "Kettle Beacon"
+ },
+ {
+ "name": "Ngobedu",
+ "pos_x": -22.34375,
+ "pos_y": -26.03125,
+ "pos_z": 50.5,
+ "stations": "Meade Installation,Morin Relay,Sharma Base"
+ },
+ {
+ "name": "Ngobogdii",
+ "pos_x": -72.625,
+ "pos_y": 46.84375,
+ "pos_z": 87.09375,
+ "stations": "Macedo Orbital,Larson Refinery"
+ },
+ {
+ "name": "Ngobokopedu",
+ "pos_x": -43.0625,
+ "pos_y": 84.65625,
+ "pos_z": -84.9375,
+ "stations": "Shiner Beacon,J. G. Ballard Prospect"
+ },
+ {
+ "name": "Ngobriglai",
+ "pos_x": 96.5625,
+ "pos_y": 6,
+ "pos_z": -9.75,
+ "stations": "Kaleri Refinery"
+ },
+ {
+ "name": "Ngobrindin",
+ "pos_x": -111.5625,
+ "pos_y": -38.34375,
+ "pos_z": -3.84375,
+ "stations": "Lubin Ring,Low Vision,Miklouho-Maclay Penal colony"
+ },
+ {
+ "name": "Ngola",
+ "pos_x": -38.125,
+ "pos_y": -146.0625,
+ "pos_z": 25.84375,
+ "stations": "Gray Station"
+ },
+ {
+ "name": "Ngolai",
+ "pos_x": -86.375,
+ "pos_y": -86.9375,
+ "pos_z": -43.84375,
+ "stations": "Hire Hub"
+ },
+ {
+ "name": "Ngolibardu",
+ "pos_x": -48.8125,
+ "pos_y": -7.75,
+ "pos_z": 39.8125,
+ "stations": "Vernadsky Port"
+ },
+ {
+ "name": "Ngolingo",
+ "pos_x": -21.15625,
+ "pos_y": 121.03125,
+ "pos_z": -129.6875,
+ "stations": "Cogswell Station,Pinto Holdings,Ozanne City,Kronecker Prospect,Bailey Installation"
+ },
+ {
+ "name": "Ngolite",
+ "pos_x": -133.78125,
+ "pos_y": -38.71875,
+ "pos_z": -26.28125,
+ "stations": "Tepper Dock,Hughes-Fulford Barracks,Moskowitz Vision"
+ },
+ {
+ "name": "Ngolitjali",
+ "pos_x": -117.53125,
+ "pos_y": 38.0625,
+ "pos_z": -4.9375,
+ "stations": "Gerst Survey"
+ },
+ {
+ "name": "Ngolock",
+ "pos_x": 141.65625,
+ "pos_y": -233.65625,
+ "pos_z": 61.125,
+ "stations": "Cortes Retreat,Tank Outpost"
+ },
+ {
+ "name": "Ngolona",
+ "pos_x": 155.03125,
+ "pos_y": -57.8125,
+ "pos_z": 79.625,
+ "stations": "Rosseland Colony,Huss Port,Stevenson Orbital,Hamilton Penal colony,Iqbal Lab"
+ },
+ {
+ "name": "Ngoma",
+ "pos_x": 71.21875,
+ "pos_y": 61.125,
+ "pos_z": 60.34375,
+ "stations": "Dyson Orbital,Duckworth Dock"
+ },
+ {
+ "name": "Ngomangatio",
+ "pos_x": 58.21875,
+ "pos_y": -232.0625,
+ "pos_z": 104.25,
+ "stations": "Ikeya's Claim,Dilworth Point"
+ },
+ {
+ "name": "Ngombivas",
+ "pos_x": 26.6875,
+ "pos_y": -35,
+ "pos_z": 75.375,
+ "stations": "Galois Enterprise,MacCurdy Port,Linaweaver Port,Norman Installation"
+ },
+ {
+ "name": "Ngones",
+ "pos_x": 98.78125,
+ "pos_y": -185.96875,
+ "pos_z": -52.75,
+ "stations": "Arisman Enterprise,Silverberg Keep,Rucker Vision"
+ },
+ {
+ "name": "Ngongani",
+ "pos_x": 195.625,
+ "pos_y": -129.6875,
+ "pos_z": 94.3125,
+ "stations": "Kurtz Station,Common Bastion,Havilland Gateway"
+ },
+ {
+ "name": "Ngonten",
+ "pos_x": -38.78125,
+ "pos_y": 110.375,
+ "pos_z": 96.5625,
+ "stations": "Serling Prospect,Drzewiecki Platform,Foden Beacon,Dyr Base"
+ },
+ {
+ "name": "Ngorowai",
+ "pos_x": 84.34375,
+ "pos_y": -86.9375,
+ "pos_z": -89.28125,
+ "stations": "Pons Gateway,Curtiss Vision,Tuttle City,Takahashi Port,Burkin Prospect,Sabine Terminal"
+ },
+ {
+ "name": "Ngugira",
+ "pos_x": 62.21875,
+ "pos_y": -24.71875,
+ "pos_z": 22.65625,
+ "stations": "Lee Port,Ockels Terminal,Bacon Market,Hurley Port,Boodt Landing,Gillekens Dock,Reiter Port,Bokeili Horizons,Bartoe Ring,Boswell Relay,Curie Terminal,Stanier City,Parazynski Point,Sanger Prospect"
+ },
+ {
+ "name": "Ngugirawa",
+ "pos_x": 11.09375,
+ "pos_y": 13.125,
+ "pos_z": 100.0625,
+ "stations": "Hadfield Enterprise,Blalock Prospect,Fraas Colony,Morgan Platform,Amundsen Barracks"
+ },
+ {
+ "name": "Ngulu",
+ "pos_x": 107.6875,
+ "pos_y": 118.59375,
+ "pos_z": -59.0625,
+ "stations": "Maudslay Dock"
+ },
+ {
+ "name": "Ngulungbara",
+ "pos_x": -1.9375,
+ "pos_y": -240.875,
+ "pos_z": 35.09375,
+ "stations": "Filippenko Dock,Flaugergues Enterprise,Chalker Settlement,Plait's Inheritance,Seitter Port"
+ },
+ {
+ "name": "Ngun",
+ "pos_x": 85.71875,
+ "pos_y": -122.625,
+ "pos_z": 8.75,
+ "stations": "Thiele Orbital,Adelman Port,Parsons Gateway"
+ },
+ {
+ "name": "Nguna",
+ "pos_x": -122.78125,
+ "pos_y": -102.53125,
+ "pos_z": -22.5625,
+ "stations": "Biggle Hub,Bear Holdings"
+ },
+ {
+ "name": "Ngunabozho",
+ "pos_x": -97.875,
+ "pos_y": -33.0625,
+ "pos_z": 95.1875,
+ "stations": "Mukai Vision,Favier Mines,Feustel Base"
+ },
+ {
+ "name": "Ngundecabui",
+ "pos_x": 144.53125,
+ "pos_y": 28.25,
+ "pos_z": -49,
+ "stations": "Harvey Horizons"
+ },
+ {
+ "name": "Ngundjedes",
+ "pos_x": -167.6875,
+ "pos_y": 69.6875,
+ "pos_z": -64.90625,
+ "stations": "Stafford Depot"
+ },
+ {
+ "name": "Nguni",
+ "pos_x": 117.15625,
+ "pos_y": -69.875,
+ "pos_z": 39.3125,
+ "stations": "Lalande Beacon"
+ },
+ {
+ "name": "Nguraci",
+ "pos_x": -47.65625,
+ "pos_y": 53.71875,
+ "pos_z": -130.34375,
+ "stations": "Rawat Settlement,Zillig Orbital,Overmyer's Folly"
+ },
+ {
+ "name": "Ngurawong",
+ "pos_x": 3.90625,
+ "pos_y": -28.4375,
+ "pos_z": 124.59375,
+ "stations": "Tsibliyev Mine,Smith's Folly"
+ },
+ {
+ "name": "Ngurd",
+ "pos_x": 6.65625,
+ "pos_y": -153.625,
+ "pos_z": 51.46875,
+ "stations": "Arago Vision,Coggia Colony"
+ },
+ {
+ "name": "Ngurelban",
+ "pos_x": -46.28125,
+ "pos_y": 21.65625,
+ "pos_z": 19.09375,
+ "stations": "Duke Hangar,Camarda Beacon,Ricardo Landing"
+ },
+ {
+ "name": "Ngurii",
+ "pos_x": 169.5,
+ "pos_y": -42.34375,
+ "pos_z": 87.0625,
+ "stations": "Cheranovsky City,Takamizawa Station,Kosai Hub"
+ },
+ {
+ "name": "Ngurlungus",
+ "pos_x": -20.875,
+ "pos_y": 99.71875,
+ "pos_z": -61.71875,
+ "stations": "Lysenko Platform,Wescott Observatory"
+ },
+ {
+ "name": "Ngurui",
+ "pos_x": 46.8125,
+ "pos_y": -106.78125,
+ "pos_z": -89.75,
+ "stations": "Hartlib Landing,Narbeth Horizons"
+ },
+ {
+ "name": "Ngurumbha",
+ "pos_x": -103.34375,
+ "pos_y": -62.625,
+ "pos_z": -47.71875,
+ "stations": "Swigert Hub,Freycinet Barracks,Wyeth Gateway,Ivanov City,Tyson Port,Phillips Dock,Salk Hub,Galilei Orbital,Chilton City,Akiyama City,Bethke Terminal,Brera Enterprise,Forward Terminal"
+ },
+ {
+ "name": "Ngurunderi",
+ "pos_x": -121.65625,
+ "pos_y": 4.40625,
+ "pos_z": 53.625,
+ "stations": "Nesvadba Platform"
+ },
+ {
+ "name": "Ngurungo",
+ "pos_x": 58.03125,
+ "pos_y": -113.375,
+ "pos_z": 43.4375,
+ "stations": "Kandrup Point"
+ },
+ {
+ "name": "Niaba",
+ "pos_x": 78.34375,
+ "pos_y": -30.65625,
+ "pos_z": 95.25,
+ "stations": "Alten Orbital,Gann Dock,Rand Station,Goldschmidt Depot,Eilenberg Terminal,Lundwall Dock"
+ },
+ {
+ "name": "Niabakanute",
+ "pos_x": 124.34375,
+ "pos_y": -137.75,
+ "pos_z": 18.90625,
+ "stations": "Mayor Orbital,Suntzeff Vision"
+ },
+ {
+ "name": "Niabi",
+ "pos_x": -48.15625,
+ "pos_y": 58.125,
+ "pos_z": 145.3125,
+ "stations": "Adams Mine"
+ },
+ {
+ "name": "Nialfi",
+ "pos_x": 109.90625,
+ "pos_y": -28.3125,
+ "pos_z": -44.59375,
+ "stations": "Artin Orbital,Paulo da Gama Barracks"
+ },
+ {
+ "name": "Niali",
+ "pos_x": 83.15625,
+ "pos_y": -191.8125,
+ "pos_z": 24.3125,
+ "stations": "Furuta Works,Israel Mines,Finlay Terminal"
+ },
+ {
+ "name": "Niamara",
+ "pos_x": 83.0625,
+ "pos_y": -10.6875,
+ "pos_z": -94.6875,
+ "stations": "Lunan Dock,Ross Dock"
+ },
+ {
+ "name": "Nian",
+ "pos_x": -97.8125,
+ "pos_y": 69.34375,
+ "pos_z": 56.46875,
+ "stations": "Bujold Settlement,Payne Bastion"
+ },
+ {
+ "name": "Nibelaako",
+ "pos_x": -150.5,
+ "pos_y": -52.9375,
+ "pos_z": -47.71875,
+ "stations": "Oramus Orbital,Scheerbart Forum,Fernandes de Queiros Hub,Schade Enterprise,Barentsz's Progress,Hogan Vista"
+ },
+ {
+ "name": "Nican",
+ "pos_x": -23.25,
+ "pos_y": -81.0625,
+ "pos_z": -122.21875,
+ "stations": "Yeliseyev Beacon,Meitner Outpost,Ramsbottom Depot"
+ },
+ {
+ "name": "Nicassee",
+ "pos_x": -20.46875,
+ "pos_y": 52.96875,
+ "pos_z": 96.5625,
+ "stations": "Barjavel Landing,Searfoss City,Herzfeld City"
+ },
+ {
+ "name": "Nicobarese",
+ "pos_x": -124.9375,
+ "pos_y": 29.90625,
+ "pos_z": -39.15625,
+ "stations": "Stephenson Ring,McKee Depot,Ramanujan Orbital,Kepler Platform"
+ },
+ {
+ "name": "Nicocheo",
+ "pos_x": 75.8125,
+ "pos_y": -220.1875,
+ "pos_z": 107.4375,
+ "stations": "Bickel Survey"
+ },
+ {
+ "name": "Nicollos",
+ "pos_x": -22.65625,
+ "pos_y": -23.5,
+ "pos_z": 98.40625,
+ "stations": "Nicollier Station,Drew Gateway,Newman Hub,Whitelaw Landing,Rawat Settlement"
+ },
+ {
+ "name": "Nicopa",
+ "pos_x": -29.53125,
+ "pos_y": -71.03125,
+ "pos_z": 164.5625,
+ "stations": "Harris Horizons,Davidson Depot"
+ },
+ {
+ "name": "Nicor",
+ "pos_x": -56.84375,
+ "pos_y": -42.71875,
+ "pos_z": 125.03125,
+ "stations": "Compton Plant,Paulo da Gama Silo"
+ },
+ {
+ "name": "Nidanga",
+ "pos_x": -31.6875,
+ "pos_y": -0.09375,
+ "pos_z": -136.34375,
+ "stations": "McDivitt Terminal,Blodgett Colony,Bishop Relay"
+ },
+ {
+ "name": "Nidarijedi",
+ "pos_x": 13.65625,
+ "pos_y": -104.90625,
+ "pos_z": -47.65625,
+ "stations": "Le Guin Arsenal,Wu Port,Rodrigues Base"
+ },
+ {
+ "name": "Nidayiman",
+ "pos_x": -10.40625,
+ "pos_y": -147.0625,
+ "pos_z": -76.09375,
+ "stations": "Messerschmitt Settlement,Kagawa Vision,Bulychev Arsenal,Lee Vision"
+ },
+ {
+ "name": "Niery",
+ "pos_x": -45.65625,
+ "pos_y": 32.9375,
+ "pos_z": -62.25,
+ "stations": "Wundt Hub"
+ },
+ {
+ "name": "Niflheimri",
+ "pos_x": 15.90625,
+ "pos_y": -38.21875,
+ "pos_z": 72.125,
+ "stations": "Roentgen Settlement"
+ },
+ {
+ "name": "Niflhel",
+ "pos_x": 68.8125,
+ "pos_y": -148.25,
+ "pos_z": 47.9375,
+ "stations": "Dyson Vision,Wilhelm von Struve Refinery,Biruni Port,Wisdom Plant,Whitford Bastion"
+ },
+ {
+ "name": "Nihal",
+ "pos_x": 98.96875,
+ "pos_y": -72.125,
+ "pos_z": -103.53125,
+ "stations": "Spedding Terminal,Fulton Landing"
+ },
+ {
+ "name": "Nihursaga",
+ "pos_x": 24.0625,
+ "pos_y": 9.3125,
+ "pos_z": -62.875,
+ "stations": "Hardwick Hub"
+ },
+ {
+ "name": "Nijolam",
+ "pos_x": 200.1875,
+ "pos_y": -107.53125,
+ "pos_z": 23.8125,
+ "stations": "Salam Orbital,Houten-Groeneveld Vision,Dumont Orbital,Bendell Prospect"
+ },
+ {
+ "name": "Nijotec",
+ "pos_x": -15,
+ "pos_y": -22.25,
+ "pos_z": 82.46875,
+ "stations": "Kizim Laboratory,Daley Plant,Porges Refinery"
+ },
+ {
+ "name": "Nijoten",
+ "pos_x": -86.9375,
+ "pos_y": -96.40625,
+ "pos_z": 67.4375,
+ "stations": "Maury Beacon,Constantine Retreat,Ore Settlement"
+ },
+ {
+ "name": "Nijotures",
+ "pos_x": 102.34375,
+ "pos_y": 132.375,
+ "pos_z": 72.3125,
+ "stations": "Pook Reach,Kroehl Camp"
+ },
+ {
+ "name": "Niju",
+ "pos_x": -42.78125,
+ "pos_y": 57.84375,
+ "pos_z": -111.75,
+ "stations": "Kingsmill Beacon,Grandin Station,Van Scyoc Enterprise"
+ },
+ {
+ "name": "Nijuhty",
+ "pos_x": 42.25,
+ "pos_y": -131.84375,
+ "pos_z": -88.28125,
+ "stations": "Ivanishin Settlement,Overmyer Silo,Creighton Enterprise"
+ },
+ {
+ "name": "Nijuna",
+ "pos_x": 41.9375,
+ "pos_y": -117.4375,
+ "pos_z": 48.21875,
+ "stations": "Potez Station,Kagawa Port,Molyneux Terminal,Friend Enterprise"
+ },
+ {
+ "name": "Nik",
+ "pos_x": 90.84375,
+ "pos_y": -20.71875,
+ "pos_z": 94.875,
+ "stations": "Noguchi Hub,Forrester Orbital,Ing Prospect,Bose Terminal,Bendell Installation"
+ },
+ {
+ "name": "Nilfheim",
+ "pos_x": 44.15625,
+ "pos_y": -7.25,
+ "pos_z": 92.40625,
+ "stations": "Allen Camp,Lopez de Legazpi Settlement"
+ },
+ {
+ "name": "Nima",
+ "pos_x": 7.5625,
+ "pos_y": 30.25,
+ "pos_z": -82.65625,
+ "stations": "Burbank Orbital,Rushworth Terminal,Cady Point,Rashid Dock,McDonald Works"
+ },
+ {
+ "name": "Niman",
+ "pos_x": -78,
+ "pos_y": -88.15625,
+ "pos_z": 42.28125,
+ "stations": "Thagard Vision,Hubble Landing,Massimino Installation"
+ },
+ {
+ "name": "Nimari",
+ "pos_x": 94.03125,
+ "pos_y": 90.6875,
+ "pos_z": 19.15625,
+ "stations": "Raleigh Settlement"
+ },
+ {
+ "name": "Nimboja",
+ "pos_x": 13.03125,
+ "pos_y": -119.75,
+ "pos_z": 134.25,
+ "stations": "Backlund Dock,Vaucouleurs Dock,Brosnatch Orbital"
+ },
+ {
+ "name": "Ninabin",
+ "pos_x": 52.125,
+ "pos_y": -51.71875,
+ "pos_z": -30.15625,
+ "stations": "Trevithick Vision,Moffitt Installation,Rushworth Colony"
+ },
+ {
+ "name": "Ninans",
+ "pos_x": -78.53125,
+ "pos_y": -55.1875,
+ "pos_z": 3.3125,
+ "stations": "Giles Survey,Hartsfield Station,Vinogradov Dock"
+ },
+ {
+ "name": "Ninhursag",
+ "pos_x": 58.125,
+ "pos_y": -44.34375,
+ "pos_z": -5.84375,
+ "stations": "Danny B Mattissen,McCoy City"
+ },
+ {
+ "name": "Ninibo",
+ "pos_x": -55.09375,
+ "pos_y": 41.53125,
+ "pos_z": -81.59375,
+ "stations": "Beebe Terminal"
+ },
+ {
+ "name": "Ninmah",
+ "pos_x": -3.625,
+ "pos_y": 1.96875,
+ "pos_z": -67.84375,
+ "stations": "Wiley Port,Gunn Base,Godwin Vista,Selberg Settlement,King Vision,Boe Stop,McCandless City,Lee Relay"
+ },
+ {
+ "name": "Ninsun",
+ "pos_x": -38.09375,
+ "pos_y": 33.40625,
+ "pos_z": 45.90625,
+ "stations": "Ramanujan Dock,Barreiros Arsenal,Abe Terminal"
+ },
+ {
+ "name": "Nipiates",
+ "pos_x": 2.28125,
+ "pos_y": -98.375,
+ "pos_z": 137.9375,
+ "stations": "Quaglia Hub,Clarke Survey,Kemurdzhian Enterprise"
+ },
+ {
+ "name": "Nipibo",
+ "pos_x": 136.59375,
+ "pos_y": -5.5625,
+ "pos_z": 4.21875,
+ "stations": "Reynolds Beacon"
+ },
+ {
+ "name": "Nipihikeggi",
+ "pos_x": 153.21875,
+ "pos_y": -123,
+ "pos_z": -64.1875,
+ "stations": "Hoffleit Platform,Kalam Retreat,Bereznyak Point"
+ },
+ {
+ "name": "Nipihila",
+ "pos_x": 69.46875,
+ "pos_y": -56.71875,
+ "pos_z": 153.375,
+ "stations": "Hatanaka Terminal,Rosenberger Installation"
+ },
+ {
+ "name": "Nipitio",
+ "pos_x": 62.5,
+ "pos_y": 2.40625,
+ "pos_z": 140.6875,
+ "stations": "Mitchell Landing"
+ },
+ {
+ "name": "Nirrayutori",
+ "pos_x": 113.6875,
+ "pos_y": -70.96875,
+ "pos_z": -81.71875,
+ "stations": "Alexandria Point"
+ },
+ {
+ "name": "Nirriti",
+ "pos_x": -54.34375,
+ "pos_y": -4.1875,
+ "pos_z": 106.9375,
+ "stations": "Potter Mines,Roosa Dock,Lefschetz Penal colony"
+ },
+ {
+ "name": "Nirrodumara",
+ "pos_x": 153.0625,
+ "pos_y": -30.625,
+ "pos_z": 58.46875,
+ "stations": "Chadwick Dock"
+ },
+ {
+ "name": "Nirros",
+ "pos_x": -62.84375,
+ "pos_y": -99.9375,
+ "pos_z": 67.46875,
+ "stations": "Littrow Enterprise"
+ },
+ {
+ "name": "Nisgan",
+ "pos_x": 93.5,
+ "pos_y": -183.1875,
+ "pos_z": 59.0625,
+ "stations": "Wachmann's Claim,Asami Prospect"
+ },
+ {
+ "name": "Nisgayo",
+ "pos_x": -18,
+ "pos_y": -59.90625,
+ "pos_z": 47.65625,
+ "stations": "Bosch City,Brunel Settlement"
+ },
+ {
+ "name": "Nithhogg",
+ "pos_x": 47.71875,
+ "pos_y": -96.25,
+ "pos_z": 117.21875,
+ "stations": "Greene Mines"
+ },
+ {
+ "name": "Nithungambo",
+ "pos_x": 95.375,
+ "pos_y": -83.40625,
+ "pos_z": 56.1875,
+ "stations": "Schwarzschild Landing,Chiang Colony"
+ },
+ {
+ "name": "Nitikaba",
+ "pos_x": 137.40625,
+ "pos_y": -75.3125,
+ "pos_z": -27.65625,
+ "stations": "Kube-McDowell Keep,Moore Port"
+ },
+ {
+ "name": "Nitikudi",
+ "pos_x": 175.46875,
+ "pos_y": -179.34375,
+ "pos_z": 41.6875,
+ "stations": "Wasden Terminal,Mizuno's Claim,Bohnhoff Holdings"
+ },
+ {
+ "name": "Nititja",
+ "pos_x": -129.8125,
+ "pos_y": -17.84375,
+ "pos_z": 45.84375,
+ "stations": "Scott's Claim,Garratt Camp"
+ },
+ {
+ "name": "Niu Anang",
+ "pos_x": -16.5625,
+ "pos_y": -89.9375,
+ "pos_z": 120.9375,
+ "stations": "Hughes Plant,Jacobi Beacon,Adams Port"
+ },
+ {
+ "name": "Niu Benzai",
+ "pos_x": -40.59375,
+ "pos_y": 44.75,
+ "pos_z": -128.1875,
+ "stations": "Midgeley Mines,Volynov Reach"
+ },
+ {
+ "name": "Niu Bese",
+ "pos_x": 67.15625,
+ "pos_y": -68.25,
+ "pos_z": -47,
+ "stations": "Krikalev Mine"
+ },
+ {
+ "name": "Niu Hsing",
+ "pos_x": 36.78125,
+ "pos_y": 155.3125,
+ "pos_z": -19.375,
+ "stations": "Cabrera's Claim,Gorbatko Terminal"
+ },
+ {
+ "name": "Niu Lang",
+ "pos_x": -61.5,
+ "pos_y": 80.28125,
+ "pos_z": -85.5625,
+ "stations": "Porsche Depot,Fischer Platform,Zoline Works"
+ },
+ {
+ "name": "Niu Lang O",
+ "pos_x": 78.5,
+ "pos_y": -93.03125,
+ "pos_z": -24.375,
+ "stations": "Hirasawa Station,Safdie Landing,Kibalchich Prospect"
+ },
+ {
+ "name": "Niu Yu",
+ "pos_x": 111.15625,
+ "pos_y": -61.75,
+ "pos_z": 8.03125,
+ "stations": "Froud Mines,Kepler Depot,McMillan Port"
+ },
+ {
+ "name": "Niu Yun",
+ "pos_x": -94.21875,
+ "pos_y": 33.4375,
+ "pos_z": -89.3125,
+ "stations": "Searfoss Vision,Langsdorff Barracks"
+ },
+ {
+ "name": "Nivati",
+ "pos_x": 111.1875,
+ "pos_y": 64,
+ "pos_z": -99.71875,
+ "stations": "Chamitoff Enterprise,Vernadsky Orbital,Frobenius Enterprise,Ashby Holdings"
+ },
+ {
+ "name": "Njamanachi",
+ "pos_x": -127,
+ "pos_y": 89.1875,
+ "pos_z": 19.75,
+ "stations": "Duffy Mine,Doi Outpost"
+ },
+ {
+ "name": "Njambalba",
+ "pos_x": -3.65625,
+ "pos_y": -153.71875,
+ "pos_z": 26.46875,
+ "stations": "Hogan's Progress,Frost Dock,Fox Vision,Pohl Prospect,Oja Settlement"
+ },
+ {
+ "name": "Njambandine",
+ "pos_x": -17.65625,
+ "pos_y": -21.125,
+ "pos_z": 86.625,
+ "stations": "Pullman Hangar,Evangelisti's Progress,Cayley Port"
+ },
+ {
+ "name": "Njambi",
+ "pos_x": 100.1875,
+ "pos_y": -73.71875,
+ "pos_z": 59,
+ "stations": "Steiner Relay,Dolgov Landing"
+ },
+ {
+ "name": "Njambian De",
+ "pos_x": 151.90625,
+ "pos_y": -118.8125,
+ "pos_z": -4.59375,
+ "stations": "Ellern Hub,White Gateway,Bass Keep"
+ },
+ {
+ "name": "Njambula",
+ "pos_x": 126.03125,
+ "pos_y": 26.21875,
+ "pos_z": -114.625,
+ "stations": "Kotzebue Terminal,Polansky Base,Sekelj Terminal"
+ },
+ {
+ "name": "Njambwa",
+ "pos_x": -74.4375,
+ "pos_y": -23.125,
+ "pos_z": 88.46875,
+ "stations": "d'Eyncourt Hub,Bordage Hangar,Payton Enterprise"
+ },
+ {
+ "name": "Njamissing",
+ "pos_x": 134,
+ "pos_y": -142.40625,
+ "pos_z": -23.09375,
+ "stations": "Stackpole Market,Bentham City,Almagro Orbital,Bixby Station,Kier Hub,Sellings Terminal,Robson City,Piazzi's Inheritance,Sitterly Bastion,Hopkinson Depot"
+ },
+ {
+ "name": "Njana",
+ "pos_x": 154.21875,
+ "pos_y": 7.625,
+ "pos_z": -78.78125,
+ "stations": "Grassmann Dock,Nobel Vision"
+ },
+ {
+ "name": "Njang",
+ "pos_x": -40.1875,
+ "pos_y": -11.875,
+ "pos_z": -141.90625,
+ "stations": "Waldeck Outpost"
+ },
+ {
+ "name": "Njangamarda",
+ "pos_x": 114.1875,
+ "pos_y": -76.40625,
+ "pos_z": -33.6875,
+ "stations": "Budrys Point,Brundage Vision,Gasparis Landing,Luu Survey"
+ },
+ {
+ "name": "Njangari",
+ "pos_x": 8.875,
+ "pos_y": -205.4375,
+ "pos_z": 64.375,
+ "stations": "Lee Hub"
+ },
+ {
+ "name": "Njanheim",
+ "pos_x": 106.8125,
+ "pos_y": -111.375,
+ "pos_z": 122.8125,
+ "stations": "Suntzeff Platform,Ziegler Hub"
+ },
+ {
+ "name": "Njanito",
+ "pos_x": -67.6875,
+ "pos_y": -84.875,
+ "pos_z": -113.9375,
+ "stations": "Czerneda Works"
+ },
+ {
+ "name": "Njemar",
+ "pos_x": 157.34375,
+ "pos_y": -204.90625,
+ "pos_z": -60.375,
+ "stations": "Fedden Stop"
+ },
+ {
+ "name": "Njemari",
+ "pos_x": 125.6875,
+ "pos_y": -33.8125,
+ "pos_z": -17.9375,
+ "stations": "Ramsbottom Station,Hughes Barracks,Edwards Platform,Stebler Port"
+ },
+ {
+ "name": "Njemayeka",
+ "pos_x": 71.34375,
+ "pos_y": -79.15625,
+ "pos_z": -76.75,
+ "stations": "Dilworth Port"
+ },
+ {
+ "name": "Njika Mo",
+ "pos_x": 93.53125,
+ "pos_y": 78.875,
+ "pos_z": -118.53125,
+ "stations": "Asaro Point"
+ },
+ {
+ "name": "Njikan",
+ "pos_x": -104.78125,
+ "pos_y": 3.65625,
+ "pos_z": -20.8125,
+ "stations": "Smeaton Port,Markov Settlement"
+ },
+ {
+ "name": "Njikas",
+ "pos_x": -139.5,
+ "pos_y": -69.96875,
+ "pos_z": 65.375,
+ "stations": "Berezin Terminal,Gunn Dock,May's Inheritance"
+ },
+ {
+ "name": "Njikit",
+ "pos_x": -138.125,
+ "pos_y": -65.4375,
+ "pos_z": 5.15625,
+ "stations": "Volkov's Progress,Pausch Landing"
+ },
+ {
+ "name": "Njiri",
+ "pos_x": 93.96875,
+ "pos_y": -96.9375,
+ "pos_z": 20.5,
+ "stations": "Korolyov Vision,Grant Terminal,Helin Dock,Andrews Terminal,Fowler Refinery"
+ },
+ {
+ "name": "Njirus",
+ "pos_x": 50.375,
+ "pos_y": -31.09375,
+ "pos_z": 4.5,
+ "stations": "Burnham Depot,Moriarty Horizons,Rocklynne Installation,Consolmagno Legacy"
+ },
+ {
+ "name": "Njoerd",
+ "pos_x": 126.84375,
+ "pos_y": -13.28125,
+ "pos_z": 75.71875,
+ "stations": "Nobel Mine"
+ },
+ {
+ "name": "Njoerdhri",
+ "pos_x": 51.5625,
+ "pos_y": -192.40625,
+ "pos_z": 105.59375,
+ "stations": "Tousey Vision,Baffin Relay"
+ },
+ {
+ "name": "Njoere",
+ "pos_x": -118.59375,
+ "pos_y": 64.03125,
+ "pos_z": -92.25,
+ "stations": "Cleave Station,Vetulani Orbital,Nehsi Laboratory,Gemar Beacon"
+ },
+ {
+ "name": "Njoeris",
+ "pos_x": -34.90625,
+ "pos_y": -26.59375,
+ "pos_z": 93.9375,
+ "stations": "Vian Installation"
+ },
+ {
+ "name": "Njokujil",
+ "pos_x": 55.75,
+ "pos_y": -93.03125,
+ "pos_z": -36.21875,
+ "stations": "Dedekind Bastion,Lindemann Dock,Palisa Ring,Lintott Gateway,Matthews Terminal,Plaskett Gateway,Arend Vision,Schlesinger City,Chandra Beacon"
+ },
+ {
+ "name": "Njokujinun",
+ "pos_x": -73.65625,
+ "pos_y": -33.03125,
+ "pos_z": -82.09375,
+ "stations": "Thurston Enterprise,Nyberg Vision,Atiyah Barracks"
+ },
+ {
+ "name": "Njordeates",
+ "pos_x": -168.75,
+ "pos_y": 53.96875,
+ "pos_z": 13.375,
+ "stations": "Madsen Point,Bierce Escape"
+ },
+ {
+ "name": "Njordi",
+ "pos_x": -109.3125,
+ "pos_y": 108.375,
+ "pos_z": -52.78125,
+ "stations": "Fontenay Station,Plexico Terminal,Lopez de Legazpi City,Martinez Laboratory"
+ },
+ {
+ "name": "Njordr",
+ "pos_x": -71.03125,
+ "pos_y": 1.28125,
+ "pos_z": 11.25,
+ "stations": "Appel City,Barry Port,Robinson Installation"
+ },
+ {
+ "name": "Njorog",
+ "pos_x": 92.5625,
+ "pos_y": -61.875,
+ "pos_z": -127.40625,
+ "stations": "Sekowski Horizons,Macgregor Orbital,Everest Dock,Sargent Base"
+ },
+ {
+ "name": "Njoror",
+ "pos_x": 120.9375,
+ "pos_y": -220.40625,
+ "pos_z": 56.96875,
+ "stations": "Suri Port,Alfven Orbital,Lichtenberg Lab,Abel Survey,Bouvard Reach"
+ },
+ {
+ "name": "Njorovices",
+ "pos_x": 35.90625,
+ "pos_y": 28.625,
+ "pos_z": -56.28125,
+ "stations": "Goldstein Ring,Cook Station,Hauck Relay,Matheson Ring"
+ },
+ {
+ "name": "Njortamo",
+ "pos_x": 134.09375,
+ "pos_y": 77.4375,
+ "pos_z": -125.46875,
+ "stations": "Maury Orbital,Gibson Arsenal,Bao Terminal,Lefschetz's Progress"
+ },
+ {
+ "name": "Njortamool",
+ "pos_x": 46.65625,
+ "pos_y": -43.34375,
+ "pos_z": -43.6875,
+ "stations": "Krikalev Hangar"
+ },
+ {
+ "name": "Njorth",
+ "pos_x": -28.40625,
+ "pos_y": -117.375,
+ "pos_z": 66.1875,
+ "stations": "MacCready City,Staus Orbital,Narita's Folly,Tanner Hub,Bok Hub"
+ },
+ {
+ "name": "Njula",
+ "pos_x": 122.625,
+ "pos_y": -163.8125,
+ "pos_z": 2.21875,
+ "stations": "Gray Terminal"
+ },
+ {
+ "name": "Njulan",
+ "pos_x": -89.625,
+ "pos_y": 47.25,
+ "pos_z": 50.59375,
+ "stations": "Romanenko Port"
+ },
+ {
+ "name": "Njulans",
+ "pos_x": 139.96875,
+ "pos_y": -171.5,
+ "pos_z": 46.46875,
+ "stations": "Elst Holdings,Michalitsianos Orbital,Baillaud Landing"
+ },
+ {
+ "name": "Njulngan",
+ "pos_x": -38.875,
+ "pos_y": -42.8125,
+ "pos_z": -90.875,
+ "stations": "Tesla Terminal,Hoshide Orbital,Wylie's Inheritance,Przhevalsky Legacy"
+ },
+ {
+ "name": "Njundji",
+ "pos_x": 95.84375,
+ "pos_y": 86.65625,
+ "pos_z": -26.84375,
+ "stations": "Pascal Terminal,Howard Enterprise,Walker Point"
+ },
+ {
+ "name": "Njunga",
+ "pos_x": 91.46875,
+ "pos_y": -32.1875,
+ "pos_z": -79.5625,
+ "stations": "Mayer Dock,Sommerfeld Terminal,Delbruck City,Binnie City,Howe Survey,Asprin Base"
+ },
+ {
+ "name": "Njungum",
+ "pos_x": 134.59375,
+ "pos_y": -21.5625,
+ "pos_z": 1.15625,
+ "stations": "Backlund Relay"
+ },
+ {
+ "name": "Njunmin",
+ "pos_x": -55.6875,
+ "pos_y": -23.90625,
+ "pos_z": -51.84375,
+ "stations": "Armstrong Platform,Daimler Enterprise,Descartes Mines"
+ },
+ {
+ "name": "Njuwai",
+ "pos_x": -21.09375,
+ "pos_y": -41.03125,
+ "pos_z": 17.15625,
+ "stations": "Bluford Vision,Wang Vision"
+ },
+ {
+ "name": "Njuwar",
+ "pos_x": 39.1875,
+ "pos_y": 12.5625,
+ "pos_z": 69.65625,
+ "stations": "Tognini Dock,Heng Hub,Cooper Hub,Boulton Station,Crown Terminal,Buckland Port,Bramah City"
+ },
+ {
+ "name": "Njuwatem",
+ "pos_x": -81.6875,
+ "pos_y": -100.5625,
+ "pos_z": 60.40625,
+ "stations": "Bates Survey,Cousteau Point"
+ },
+ {
+ "name": "Nkan",
+ "pos_x": 42.6875,
+ "pos_y": 3.40625,
+ "pos_z": -166.125,
+ "stations": "Rochon Camp,Killough Bastion"
+ },
+ {
+ "name": "Nkana",
+ "pos_x": 43.875,
+ "pos_y": 143.625,
+ "pos_z": 6.21875,
+ "stations": "Chadwick Dock,McBride Refinery"
+ },
+ {
+ "name": "Nkanu",
+ "pos_x": 66.9375,
+ "pos_y": 18.84375,
+ "pos_z": -129.9375,
+ "stations": "Regiomontanus Enterprise,Aldiss Dock,Bunch Port,Roberts Depot"
+ },
+ {
+ "name": "Nkonang",
+ "pos_x": 133.1875,
+ "pos_y": -2.71875,
+ "pos_z": 63.5,
+ "stations": "Cugnot Refinery,Swigert Plant"
+ },
+ {
+ "name": "Nkonjurungu",
+ "pos_x": -81.65625,
+ "pos_y": -80.375,
+ "pos_z": 19.625,
+ "stations": "Perrin Escape,Hieb Platform"
+ },
+ {
+ "name": "Nkonoman",
+ "pos_x": -42.4375,
+ "pos_y": 151.5,
+ "pos_z": 20.9375,
+ "stations": "Shaw Mines,Janifer's Folly"
+ },
+ {
+ "name": "Nkwalas",
+ "pos_x": -8.3125,
+ "pos_y": 50.25,
+ "pos_z": -113.75,
+ "stations": "McCulley Hub,Parazynski Hub,Fung Terminal,Trevithick Keep"
+ },
+ {
+ "name": "NLTT 10055",
+ "pos_x": 84.1875,
+ "pos_y": -108.9375,
+ "pos_z": 1.90625,
+ "stations": "Barnwell Landing,Goodman Station"
+ },
+ {
+ "name": "NLTT 1139",
+ "pos_x": -64.9375,
+ "pos_y": -26.5,
+ "pos_z": -33.40625,
+ "stations": "Oluwafemi Works"
+ },
+ {
+ "name": "NLTT 11599",
+ "pos_x": 48.78125,
+ "pos_y": -62.09375,
+ "pos_z": -11.71875,
+ "stations": "Babakin Terminal,Young Works,Tombaugh Port,Janjetov City,Ellison Installation"
+ },
+ {
+ "name": "NLTT 12130",
+ "pos_x": 1.75,
+ "pos_y": -63.59375,
+ "pos_z": -99.65625,
+ "stations": "Hodgkinson Colony,Rolland Settlement"
+ },
+ {
+ "name": "NLTT 12619",
+ "pos_x": 0.5,
+ "pos_y": -48.75,
+ "pos_z": -93.90625,
+ "stations": "Wyeth Refinery,Rangarajan Barracks"
+ },
+ {
+ "name": "NLTT 13249",
+ "pos_x": -38.8125,
+ "pos_y": 9.3125,
+ "pos_z": -60.53125,
+ "stations": "Gibbs Enterprise,Harper Platform,Lewis Orbital,Tokubei Base"
+ },
+ {
+ "name": "NLTT 14641",
+ "pos_x": 97.625,
+ "pos_y": -65.1875,
+ "pos_z": 35.03125,
+ "stations": "Brashear Refinery"
+ },
+ {
+ "name": "NLTT 14879",
+ "pos_x": -31.71875,
+ "pos_y": 14,
+ "pos_z": -67.5,
+ "stations": "Crown Camp,Kennan Vision,Vries Base"
+ },
+ {
+ "name": "NLTT 1512",
+ "pos_x": -24.1875,
+ "pos_y": -113.875,
+ "pos_z": -3.84375,
+ "stations": "Schlesinger City,Guth Plant,Mawson's Folly"
+ },
+ {
+ "name": "NLTT 15714",
+ "pos_x": 15.40625,
+ "pos_y": -8.375,
+ "pos_z": -31.5,
+ "stations": "Ivanchenkov Port,Pascal Plant"
+ },
+ {
+ "name": "NLTT 16389",
+ "pos_x": -13.3125,
+ "pos_y": 18.1875,
+ "pos_z": -74.34375,
+ "stations": "Wrangell Dock,Eilenberg Oasis,Smith Stop"
+ },
+ {
+ "name": "NLTT 16391",
+ "pos_x": 42.90625,
+ "pos_y": -8.65625,
+ "pos_z": -81.875,
+ "stations": "Bisson Dock,Nourse Installation,Williamson Vision"
+ },
+ {
+ "name": "NLTT 16570",
+ "pos_x": 40.1875,
+ "pos_y": 1.34375,
+ "pos_z": -104.59375,
+ "stations": "Kozeyev Ring,Vela City,Hoften Dock,Gunn Arsenal"
+ },
+ {
+ "name": "NLTT 18561",
+ "pos_x": 43.96875,
+ "pos_y": 23.09375,
+ "pos_z": -64.6875,
+ "stations": "Bowersox Station,Wilcutt Terminal,Broglie Vision,Euclid Depot,Duckworth Enterprise,Manarov Refinery"
+ },
+ {
+ "name": "NLTT 18945",
+ "pos_x": 3.84375,
+ "pos_y": 30.375,
+ "pos_z": -52.0625,
+ "stations": "Houtman Ring,Kelleam Station,Kneale Refinery,Ibold Hub,Alenquer City,Crown Gateway"
+ },
+ {
+ "name": "NLTT 19375",
+ "pos_x": 105.71875,
+ "pos_y": -33.625,
+ "pos_z": 21.625,
+ "stations": "Mayor Port,Wiener Vision"
+ },
+ {
+ "name": "NLTT 19808",
+ "pos_x": 46.09375,
+ "pos_y": 26.78125,
+ "pos_z": -43.71875,
+ "stations": "Maxwell Market,Ford Dock,Ham Terminal,Haisheng Dock,Lopez de Haro Colony,Bardeen Dock,Francisco de Eliza Holdings,Volynov's Inheritance"
+ },
+ {
+ "name": "NLTT 20330",
+ "pos_x": -13.9375,
+ "pos_y": 44.71875,
+ "pos_z": -53.21875,
+ "stations": "Russell Station,Asprin Terminal,Card Penal colony,Leopold Works"
+ },
+ {
+ "name": "NLTT 21088",
+ "pos_x": 71.5625,
+ "pos_y": 11.375,
+ "pos_z": -12.5,
+ "stations": "Weber Terminal,Dobrovolski Ring,Hertz Station"
+ },
+ {
+ "name": "NLTT 21176",
+ "pos_x": -6.6875,
+ "pos_y": 55.625,
+ "pos_z": -58.0625,
+ "stations": "Conway Dock,Cook Dock,Franklin Stop,Wait Colburn Installation"
+ },
+ {
+ "name": "NLTT 21440",
+ "pos_x": 3.59375,
+ "pos_y": 46.1875,
+ "pos_z": -46.65625,
+ "stations": "Higginbotham Landing,Delbruck Gateway,Manning Vista,Drexler Works,Gooch Gateway,Detmer Beacon,Soddy Relay,Barr Reach,Winne Observatory"
+ },
+ {
+ "name": "NLTT 23036",
+ "pos_x": 22.34375,
+ "pos_y": 61.53125,
+ "pos_z": -44.5,
+ "stations": "Locke Works,Gregory Colony,Hilbert Arena"
+ },
+ {
+ "name": "NLTT 25207",
+ "pos_x": 83.71875,
+ "pos_y": 34.375,
+ "pos_z": 7.65625,
+ "stations": "Vlamingh Installation,Bernoulli Port"
+ },
+ {
+ "name": "NLTT 26167",
+ "pos_x": 56.53125,
+ "pos_y": 101.6875,
+ "pos_z": -25.3125,
+ "stations": "Walheim Works"
+ },
+ {
+ "name": "NLTT 2682",
+ "pos_x": 69.46875,
+ "pos_y": -164.59375,
+ "pos_z": 45.625,
+ "stations": "Yerka Colony,Kempf Terminal,Folland Vision"
+ },
+ {
+ "name": "NLTT 27413",
+ "pos_x": 25.875,
+ "pos_y": 103.8125,
+ "pos_z": -25.84375,
+ "stations": "Hilbert Hub,Lee Keep,Santarem Dock,Nelson Ring"
+ },
+ {
+ "name": "NLTT 2969",
+ "pos_x": -69.5625,
+ "pos_y": -25.0625,
+ "pos_z": -46.71875,
+ "stations": "Francisco de Almeida Port,MacLeod City,Newcomen Installation,Ross Hub,Larson Terminal"
+ },
+ {
+ "name": "NLTT 33782",
+ "pos_x": 18.84375,
+ "pos_y": 111.3125,
+ "pos_z": 31.1875,
+ "stations": "Nearchus City,Cobb Gateway,Alexandria Terminal"
+ },
+ {
+ "name": "NLTT 34715",
+ "pos_x": -27.4375,
+ "pos_y": 66.53125,
+ "pos_z": -5.65625,
+ "stations": "McAllaster Terminal,Litke Orbital,Mouhot Orbital,von Krusenstern Silo"
+ },
+ {
+ "name": "NLTT 34755",
+ "pos_x": 26,
+ "pos_y": 62.375,
+ "pos_z": 36.59375,
+ "stations": "Leopold Terminal,Deere Ring"
+ },
+ {
+ "name": "NLTT 34960",
+ "pos_x": 57.78125,
+ "pos_y": 72.125,
+ "pos_z": 66.96875,
+ "stations": "Comino Gateway,Shunn Laboratory,Kroehl Depot,Moseley Colony,Wakata Dock"
+ },
+ {
+ "name": "NLTT 35146",
+ "pos_x": 86.65625,
+ "pos_y": 9.75,
+ "pos_z": 74.9375,
+ "stations": "Henry Ring,Clement Ring,Stafford Camp,Thirsk Ring,Bao Hub,Edwards Station,Pogue Orbital,Houtman Vista,Planck Terminal"
+ },
+ {
+ "name": "NLTT 36689",
+ "pos_x": 44.125,
+ "pos_y": 17.84375,
+ "pos_z": 50.1875,
+ "stations": "Francisco de Eliza Enterprise,Burkin Lab"
+ },
+ {
+ "name": "NLTT 38960",
+ "pos_x": 63.3125,
+ "pos_y": 25.96875,
+ "pos_z": 95.84375,
+ "stations": "Sarafanov Orbital,Kerwin Dock,Leavitt Enterprise,Bowen Terminal,Higginbotham Station,Sarmiento de Gamboa Vista,Malaspina Vision,Springer Installation"
+ },
+ {
+ "name": "NLTT 40378",
+ "pos_x": 72.3125,
+ "pos_y": -27.1875,
+ "pos_z": 68.8125,
+ "stations": "Adams Enterprise,Ramsbottom Orbital,Hopper Landing"
+ },
+ {
+ "name": "NLTT 41463",
+ "pos_x": -64.75,
+ "pos_y": 70.5625,
+ "pos_z": 6.5,
+ "stations": "Levy Port,Gaultier de Varennes Dock,Varthema Terminal,Manning City,Amnuel City,Galiano Orbital"
+ },
+ {
+ "name": "NLTT 41712",
+ "pos_x": -12,
+ "pos_y": 57.625,
+ "pos_z": 79.75,
+ "stations": "Burstein Landing,Keyes Port,Piercy Installation"
+ },
+ {
+ "name": "NLTT 42544",
+ "pos_x": -73.75,
+ "pos_y": 72.53125,
+ "pos_z": 13.1875,
+ "stations": "Kummer Arsenal,Burroughs Colony"
+ },
+ {
+ "name": "NLTT 44018",
+ "pos_x": -28.34375,
+ "pos_y": 32.8125,
+ "pos_z": 54.15625,
+ "stations": "Rontgen Station"
+ },
+ {
+ "name": "NLTT 44219",
+ "pos_x": -20.5625,
+ "pos_y": 23.375,
+ "pos_z": 47.65625,
+ "stations": "Rothfuss' Pride,Benford Dock,Precourt Hub,Farkas Base"
+ },
+ {
+ "name": "NLTT 44958",
+ "pos_x": -58.65625,
+ "pos_y": 39.28125,
+ "pos_z": 17.09375,
+ "stations": "Anderson Orbital"
+ },
+ {
+ "name": "NLTT 45090",
+ "pos_x": -87.625,
+ "pos_y": 57.1875,
+ "pos_z": 26.3125,
+ "stations": "Tyson Survey"
+ },
+ {
+ "name": "NLTT 45576",
+ "pos_x": -45.71875,
+ "pos_y": 25.65625,
+ "pos_z": 54.8125,
+ "stations": "Kozlov Keep,vo Refinery,Kadenyuk Outpost"
+ },
+ {
+ "name": "NLTT 46403",
+ "pos_x": -101.71875,
+ "pos_y": 43.46875,
+ "pos_z": 45.71875,
+ "stations": "Kovalevsky Dock,Davis Hub,Wrangell's Claim"
+ },
+ {
+ "name": "NLTT 46621",
+ "pos_x": -43.8125,
+ "pos_y": 20.34375,
+ "pos_z": 4.8125,
+ "stations": "Nusslein-Volhard Terminal,Wedge Market,King Arsenal,Lounge Relay"
+ },
+ {
+ "name": "NLTT 46709",
+ "pos_x": -65.3125,
+ "pos_y": 25.875,
+ "pos_z": 23.6875,
+ "stations": "Perrin Settlement,Chiao Stop"
+ },
+ {
+ "name": "NLTT 4671",
+ "pos_x": 69.34375,
+ "pos_y": -96.125,
+ "pos_z": 36.125,
+ "stations": "Kawanishi Platform,Lyne Terminal"
+ },
+ {
+ "name": "NLTT 47967",
+ "pos_x": -32.09375,
+ "pos_y": -34.53125,
+ "pos_z": 93.03125,
+ "stations": "Tereshkova Orbital,Normand Landing,Barth Keep,Hernandez Orbital,Asher Vision"
+ },
+ {
+ "name": "NLTT 48502",
+ "pos_x": -99.5625,
+ "pos_y": 27.125,
+ "pos_z": -4.25,
+ "stations": "Onnes Colony,Oltion Lab,Drexler Dock"
+ },
+ {
+ "name": "NLTT 49528",
+ "pos_x": -72.625,
+ "pos_y": 18.5,
+ "pos_z": -11.75,
+ "stations": "O'Connor Landing,Besonders Arena,Titov Settlement,Sargent's Folly,Anning Hub"
+ },
+ {
+ "name": "NLTT 50716",
+ "pos_x": -90.96875,
+ "pos_y": 40.40625,
+ "pos_z": -42.0625,
+ "stations": "Halsell Port,Kubasov Colony,Roberts Barracks"
+ },
+ {
+ "name": "NLTT 51200",
+ "pos_x": -62.5625,
+ "pos_y": -38.9375,
+ "pos_z": 34.96875,
+ "stations": "Strekalov Colony,Vian Works"
+ },
+ {
+ "name": "NLTT 51966",
+ "pos_x": -50.40625,
+ "pos_y": -37.875,
+ "pos_z": 25.3125,
+ "stations": "Roosa Port,Erikson Landing,Ogden Silo"
+ },
+ {
+ "name": "NLTT 52560",
+ "pos_x": -98.75,
+ "pos_y": -15.5625,
+ "pos_z": -5.25,
+ "stations": "Krylov Beacon,Jemison Point,Gresley Orbital,Robinson Port,Klimuk Terminal"
+ },
+ {
+ "name": "NLTT 5369",
+ "pos_x": -54.90625,
+ "pos_y": -67.40625,
+ "pos_z": -59.8125,
+ "stations": "Redi Terminal,Coelho Beacon,Melvin Plant"
+ },
+ {
+ "name": "NLTT 53690",
+ "pos_x": -64.5625,
+ "pos_y": -29.21875,
+ "pos_z": 3.21875,
+ "stations": "Vinci Ring,Goddard Enterprise,Stapledon Landing,Levi-Montalcini Ring,Garan Ring,Thagard Terminal,Fossey City,Foucault Terminal,Beliaev Enterprise,Planck Port,Piercy Holdings,Melroy Enterprise,Rasch Survey"
+ },
+ {
+ "name": "NLTT 53889",
+ "pos_x": -76.5,
+ "pos_y": 7.6875,
+ "pos_z": -24.96875,
+ "stations": "Perga Base,Carpini Vision,Holberg Hub,Pordenone Port"
+ },
+ {
+ "name": "NLTT 55164",
+ "pos_x": -13.375,
+ "pos_y": -21.65625,
+ "pos_z": 6.59375,
+ "stations": "Martinez Depot,Kafka Installation,Caryanda Point"
+ },
+ {
+ "name": "NLTT 56379",
+ "pos_x": 8.5,
+ "pos_y": -97.53125,
+ "pos_z": 42.0625,
+ "stations": "Xin Hub,Bruck Ring,Korolyov's Folly,Sargent Hub"
+ },
+ {
+ "name": "NLTT 56881",
+ "pos_x": -108.6875,
+ "pos_y": -30.40625,
+ "pos_z": -35.71875,
+ "stations": "Pascal Hub,Irvin Enterprise,Chu Enterprise,Linnehan Hub,Schweickart Orbital,Heng Port,Balandin Ring,Sullivan Ring,Mach Hub,Hunziker's Inheritance,Bolger Keep,Ellison Prospect,Bogdanov's Inheritance"
+ },
+ {
+ "name": "NLTT 57216",
+ "pos_x": -102.96875,
+ "pos_y": -34.53125,
+ "pos_z": -34.59375,
+ "stations": "Cenker Hub,Rashid Orbital,Buckey Ring,Fourneyron Orbital,Carlisle Enterprise,Wingqvist Terminal,Guest Station,England City,Laliberte Dock,Morgan Works,Kube-McDowell Arsenal"
+ },
+ {
+ "name": "NLTT 57668",
+ "pos_x": 23.46875,
+ "pos_y": -79.65625,
+ "pos_z": 34.34375,
+ "stations": "Walotsky Landing,Moran Bastion,Ban Terminal"
+ },
+ {
+ "name": "NLTT 5871",
+ "pos_x": 30.71875,
+ "pos_y": -100.3125,
+ "pos_z": -3.40625,
+ "stations": "Valz Survey,von Zach Outpost,Regiomontanus' Inheritance"
+ },
+ {
+ "name": "NLTT 58744",
+ "pos_x": 58.09375,
+ "pos_y": -156.375,
+ "pos_z": 63.34375,
+ "stations": "Denning Vision,Dassault Dock,Hanke-Woods Landing,Ziljak Camp,Flettner Point"
+ },
+ {
+ "name": "NLTT 639",
+ "pos_x": -79.15625,
+ "pos_y": -78.625,
+ "pos_z": -31.21875,
+ "stations": "Wilson Orbital"
+ },
+ {
+ "name": "NLTT 6655",
+ "pos_x": -52.90625,
+ "pos_y": -23.78125,
+ "pos_z": -54.46875,
+ "stations": "Kotov Enterprise,Hartsfield Gateway,Jahn Survey,Good Hub"
+ },
+ {
+ "name": "NLTT 6667",
+ "pos_x": -49.8125,
+ "pos_y": -33.4375,
+ "pos_z": -55.28125,
+ "stations": "Richards Survey,Gurragchaa's Folly,Yeliseyev Port,Powers Survey"
+ },
+ {
+ "name": "NLTT 7789",
+ "pos_x": 76.75,
+ "pos_y": -97.15625,
+ "pos_z": 24.28125,
+ "stations": "Roed Odegaard Mines,Gutierrez Mines,Asaro Prison"
+ },
+ {
+ "name": "NLTT 7856",
+ "pos_x": 72.125,
+ "pos_y": -120.71875,
+ "pos_z": 6.84375,
+ "stations": "Hussey Survey,Emshwiller Dock,Flettner Vision"
+ },
+ {
+ "name": "NLTT 8084",
+ "pos_x": -11,
+ "pos_y": -60.6875,
+ "pos_z": -44.1875,
+ "stations": "Surayev Hub,Cockrell Terminal,Meucci Landing,Grimwood Settlement"
+ },
+ {
+ "name": "NLTT 8234",
+ "pos_x": 17,
+ "pos_y": -103.71875,
+ "pos_z": -44.1875,
+ "stations": "Artsutanov Orbital,Flettner Vision,Thierree Park,Tiedemann Relay"
+ },
+ {
+ "name": "NLTT 8653",
+ "pos_x": 44.15625,
+ "pos_y": -101.03125,
+ "pos_z": -20.03125,
+ "stations": "Seamans Landing,Marriott Station,McDermott Arsenal"
+ },
+ {
+ "name": "NLTT 8662",
+ "pos_x": -7.71875,
+ "pos_y": -58.90625,
+ "pos_z": -47.46875,
+ "stations": "Meucci Mine,Torricelli Enterprise"
+ },
+ {
+ "name": "NLTT 897",
+ "pos_x": 57.25,
+ "pos_y": -162.25,
+ "pos_z": 54.90625,
+ "stations": "Hoffleit Platform"
+ },
+ {
+ "name": "NLTT 9032",
+ "pos_x": -6.625,
+ "pos_y": -80.625,
+ "pos_z": -66.65625,
+ "stations": "Tapinas Hub,Edwards Refinery,Hunziker Landing"
+ },
+ {
+ "name": "NLTT 9447",
+ "pos_x": 32.625,
+ "pos_y": -96.75,
+ "pos_z": -41.5,
+ "stations": "Kozin Lab,Picacio Station,Seyfert Port,Bruce Stop,Rubin Terminal,Royo Dock,Brom Orbital,Cerulli City,Dalgarno City,Gaspar de Portola Hub,Bharadwaj Installation,Mourelle Settlement,Fabricius Vision,Zetford Enterprise,Sheffield Holdings,Puiseux Terminal"
+ },
+ {
+ "name": "NLTT 9949",
+ "pos_x": 45.53125,
+ "pos_y": -53.9375,
+ "pos_z": 5.5625,
+ "stations": "Gropple,Alternation,Szilard Vista,Skripochka Market"
+ },
+ {
+ "name": "NN 3062",
+ "pos_x": -62.8125,
+ "pos_y": -145.71875,
+ "pos_z": -37.6875,
+ "stations": "Somayaji Port,Youll Refinery"
+ },
+ {
+ "name": "NN 3365",
+ "pos_x": -18.46875,
+ "pos_y": 11.5625,
+ "pos_z": -80.3125,
+ "stations": "Hopper Orbital,Polyakov Ring,MacLeod Point,Mendel Holdings,Brandenstein's Inheritance"
+ },
+ {
+ "name": "No Cha",
+ "pos_x": 72.15625,
+ "pos_y": -191.625,
+ "pos_z": 23.59375,
+ "stations": "de Seversky Port,Roe Orbital,Frost Enterprise,Snodgrass' Progress"
+ },
+ {
+ "name": "No Chang",
+ "pos_x": 66.5625,
+ "pos_y": -44.1875,
+ "pos_z": -120.625,
+ "stations": "Koch Terminal"
+ },
+ {
+ "name": "No Chianga",
+ "pos_x": 56.59375,
+ "pos_y": 72.375,
+ "pos_z": -63.09375,
+ "stations": "Przhevalsky Port,Remec Dock,Cady City,Shinn Port,Clayton Works,Sheffield Terminal,Vercors Survey,Barcelos Base,Thornycroft Landing"
+ },
+ {
+ "name": "No Chique",
+ "pos_x": -117.46875,
+ "pos_y": 24.46875,
+ "pos_z": -29.8125,
+ "stations": "Musa al-Khwarizmi Port,Dorsett Vision"
+ },
+ {
+ "name": "No Choen",
+ "pos_x": -1.21875,
+ "pos_y": 31.75,
+ "pos_z": 172.9375,
+ "stations": "Akers Dock"
+ },
+ {
+ "name": "No Kono",
+ "pos_x": -96.09375,
+ "pos_y": -94.875,
+ "pos_z": 64.21875,
+ "stations": "Planck Depot,Gardner Relay"
+ },
+ {
+ "name": "No Miki",
+ "pos_x": 38.03125,
+ "pos_y": -104.90625,
+ "pos_z": 139.03125,
+ "stations": "Chadwick Dock"
+ },
+ {
+ "name": "No Mina",
+ "pos_x": 111.3125,
+ "pos_y": 9.84375,
+ "pos_z": 8.46875,
+ "stations": "Avicenna Port,Volynov Refinery,Durrance Enterprise"
+ },
+ {
+ "name": "No Myoin",
+ "pos_x": -133.5,
+ "pos_y": 15.90625,
+ "pos_z": 120.65625,
+ "stations": "Bounds Terminal,Bass Park,Poincare Platform,Ford Landing"
+ },
+ {
+ "name": "No Myoino",
+ "pos_x": -12.90625,
+ "pos_y": -49.0625,
+ "pos_z": 161.15625,
+ "stations": "Kolmogorov Mines"
+ },
+ {
+ "name": "Noanama",
+ "pos_x": -97.34375,
+ "pos_y": -5.96875,
+ "pos_z": 87.78125,
+ "stations": "Hartog Dock,Park Escape"
+ },
+ {
+ "name": "Noati",
+ "pos_x": 115.8125,
+ "pos_y": -120.5,
+ "pos_z": 16.96875,
+ "stations": "Seyfert Gateway,McIntosh Port,Brom Terminal,Bugrov Terminal,Lopez Landing"
+ },
+ {
+ "name": "Noatiaca",
+ "pos_x": -17,
+ "pos_y": 8.3125,
+ "pos_z": 61.34375,
+ "stations": "Bacon Survey,Haisheng Orbital,Savery Camp,Khayyam Beacon,Euclid Holdings"
+ },
+ {
+ "name": "Noatin",
+ "pos_x": 132.84375,
+ "pos_y": -1.78125,
+ "pos_z": 87.875,
+ "stations": "Lobachevsky Survey,Godel Plant"
+ },
+ {
+ "name": "Noatine",
+ "pos_x": -35.0625,
+ "pos_y": 31.46875,
+ "pos_z": 103.125,
+ "stations": "Dias Beacon,Panshin Base"
+ },
+ {
+ "name": "Noatir",
+ "pos_x": 60.40625,
+ "pos_y": 83.5,
+ "pos_z": 83.875,
+ "stations": "Bosch Terminal,Dantec Relay"
+ },
+ {
+ "name": "Noco",
+ "pos_x": -49.65625,
+ "pos_y": -64.5625,
+ "pos_z": 152.875,
+ "stations": "Jahn Dock,Fontana Orbital"
+ },
+ {
+ "name": "Nocoma",
+ "pos_x": 41.3125,
+ "pos_y": 48.71875,
+ "pos_z": 67.5,
+ "stations": "Boe Vision,Tani Mines,Barth Prison"
+ },
+ {
+ "name": "Nocopani",
+ "pos_x": 148.0625,
+ "pos_y": -48.09375,
+ "pos_z": 110.78125,
+ "stations": "Luther Orbital,DiFate Platform,Korolyov's Inheritance,Antonio de Andrade Horizons"
+ },
+ {
+ "name": "Nocori",
+ "pos_x": -32.875,
+ "pos_y": -33.46875,
+ "pos_z": 72.65625,
+ "stations": "Sadi Carnot Prospect,Bramah Station"
+ },
+ {
+ "name": "Nocorioson",
+ "pos_x": 59.5,
+ "pos_y": 18.53125,
+ "pos_z": -87.65625,
+ "stations": "Kerwin Plant,Napier Plant,Bridges Platform"
+ },
+ {
+ "name": "Noctero",
+ "pos_x": 60.96875,
+ "pos_y": -168.15625,
+ "pos_z": 129.4375,
+ "stations": "Goodricke Dock,Flaugergues Terminal,Pei Station"
+ },
+ {
+ "name": "Noegin",
+ "pos_x": -42.53125,
+ "pos_y": -38.0625,
+ "pos_z": 44.3125,
+ "stations": "Burton Escape"
+ },
+ {
+ "name": "Nogais",
+ "pos_x": 40.53125,
+ "pos_y": -144.96875,
+ "pos_z": 87.6875,
+ "stations": "van Royen Dock,Froud City,Tyson Dock,Schmidt Settlement,Matthews Survey"
+ },
+ {
+ "name": "Nogambe",
+ "pos_x": 121.40625,
+ "pos_y": 9.40625,
+ "pos_z": -76,
+ "stations": "Bunnell Dock,Hudson Arsenal,Svavarsson Orbital"
+ },
+ {
+ "name": "Nogatas",
+ "pos_x": 15.90625,
+ "pos_y": -99.625,
+ "pos_z": -39.15625,
+ "stations": "Wilhelm von Struve Dock,Gorgani Platform,Toll Base,Wolfe Point,Hoshi's Progress,Trumpler Vision"
+ },
+ {
+ "name": "Nogatyanka",
+ "pos_x": 175.09375,
+ "pos_y": -146.65625,
+ "pos_z": 69.34375,
+ "stations": "Pogson Station,Macomb Terminal,Wegner Terminal,Thomas Landing"
+ },
+ {
+ "name": "Nohocherth",
+ "pos_x": -10.0625,
+ "pos_y": -69.3125,
+ "pos_z": 120.21875,
+ "stations": "Amis Beacon,Stephens Prospect"
+ },
+ {
+ "name": "Nohock Ek",
+ "pos_x": -85.65625,
+ "pos_y": 40.8125,
+ "pos_z": 28.03125,
+ "stations": "Trinh Prospect,Virts Landing,Lovell Installation"
+ },
+ {
+ "name": "Nokana",
+ "pos_x": 108.84375,
+ "pos_y": -67.9375,
+ "pos_z": -80.78125,
+ "stations": "Wafer Settlement,Courvoisier Prospect"
+ },
+ {
+ "name": "Nokomis",
+ "pos_x": 56.90625,
+ "pos_y": -21.6875,
+ "pos_z": -37.21875,
+ "stations": "Gregory Orbital,Lee Port,Chamitoff City,Hadamard Installation,Kregel Vision"
+ },
+ {
+ "name": "Nokomishi",
+ "pos_x": -55.375,
+ "pos_y": -100.59375,
+ "pos_z": -39.03125,
+ "stations": "Bulleid Hub,Cabrera Terminal,Birkeland Works"
+ },
+ {
+ "name": "Nokonda",
+ "pos_x": -117.15625,
+ "pos_y": -78.1875,
+ "pos_z": -39.84375,
+ "stations": "Wakata Platform"
+ },
+ {
+ "name": "Nokotaikuyu",
+ "pos_x": 78.21875,
+ "pos_y": 129.5625,
+ "pos_z": 56.71875,
+ "stations": "Townshend Port,Fermi Orbital,Janszoon Base"
+ },
+ {
+ "name": "Nomm Cha",
+ "pos_x": 82.78125,
+ "pos_y": -5.9375,
+ "pos_z": -18.8125,
+ "stations": "Whymper Plant"
+ },
+ {
+ "name": "Nommai",
+ "pos_x": 142.0625,
+ "pos_y": 62.84375,
+ "pos_z": -80.875,
+ "stations": "Kuttner Installation,Anderson Port,Doyle Orbital,White Terminal,Fibonacci Point"
+ },
+ {
+ "name": "Nommamalanu",
+ "pos_x": 96.84375,
+ "pos_y": -32.09375,
+ "pos_z": -94.90625,
+ "stations": "Revin Landing,Anthony Prospect,Mohri Relay"
+ },
+ {
+ "name": "Nommarini",
+ "pos_x": -77.875,
+ "pos_y": -8.34375,
+ "pos_z": -80.3125,
+ "stations": "Peterson Point"
+ },
+ {
+ "name": "Nona",
+ "pos_x": 5.28125,
+ "pos_y": -33.28125,
+ "pos_z": 47.75,
+ "stations": "Davis Terminal,Novitski City,Khan City,Thomas Port,Ordway Arsenal,Bennett Gateway,Mallory's Folly,Savinykh Orbital,Laveykin Terminal,Broglie Dock,Roentgen Port,Schirra's Inheritance,Fermi Holdings,Brera Horizons"
+ },
+ {
+ "name": "Nones",
+ "pos_x": -136.84375,
+ "pos_y": 78.28125,
+ "pos_z": -69.84375,
+ "stations": "Daniel Port,Noon Enterprise,Forbes Colony"
+ },
+ {
+ "name": "Noneskit",
+ "pos_x": 95.78125,
+ "pos_y": -27.71875,
+ "pos_z": 100.75,
+ "stations": "Cousteau Sanctuary"
+ },
+ {
+ "name": "Noongaa",
+ "pos_x": 8.75,
+ "pos_y": -3.3125,
+ "pos_z": -173.15625,
+ "stations": "Goonan Orbital,Lehtonen Beacon,Stevin Colony"
+ },
+ {
+ "name": "Noongatiiq",
+ "pos_x": -20.28125,
+ "pos_y": -99.9375,
+ "pos_z": -132.15625,
+ "stations": "King Outpost"
+ },
+ {
+ "name": "Noonitokomu",
+ "pos_x": 1.40625,
+ "pos_y": -88.09375,
+ "pos_z": 70.9375,
+ "stations": "Wallis Mine"
+ },
+ {
+ "name": "Nootaitya",
+ "pos_x": 135.4375,
+ "pos_y": 59.0625,
+ "pos_z": 46.40625,
+ "stations": "Verne Outpost"
+ },
+ {
+ "name": "Nootayuto",
+ "pos_x": 61.875,
+ "pos_y": 159.4375,
+ "pos_z": -69.0625,
+ "stations": "Glashow Colony,Adamson Port"
+ },
+ {
+ "name": "Nootkena",
+ "pos_x": -103.875,
+ "pos_y": 0.9375,
+ "pos_z": -78.46875,
+ "stations": "Boulton Port,Planck Station,Hoyle Installation"
+ },
+ {
+ "name": "Nootkenk",
+ "pos_x": -66,
+ "pos_y": 41.84375,
+ "pos_z": 15.34375,
+ "stations": "Zettel Settlement"
+ },
+ {
+ "name": "Nora",
+ "pos_x": 92.65625,
+ "pos_y": -213,
+ "pos_z": 82.0625,
+ "stations": "Lee Orbital,Hoffleit Colony,Gotz Dock,Korolyov Settlement"
+ },
+ {
+ "name": "Norn",
+ "pos_x": 14.75,
+ "pos_y": -39.625,
+ "pos_z": -77.59375,
+ "stations": "DeLucas Enterprise,Brongniart Port,Gubarev Enterprise,Pettit's Inheritance,Clark Terminal,Armstrong Dock,Herjulfsson Holdings"
+ },
+ {
+ "name": "Nornari",
+ "pos_x": 4.5625,
+ "pos_y": -2.78125,
+ "pos_z": 45.375,
+ "stations": "Semeonis Orbital,Klein Orbital,Carstensz Settlement"
+ },
+ {
+ "name": "Nortes",
+ "pos_x": 69.53125,
+ "pos_y": -157.0625,
+ "pos_z": 54.53125,
+ "stations": "Mikoyan Hub,Hobbes Hope,Ash's Excelsis"
+ },
+ {
+ "name": "Norwar",
+ "pos_x": 88.625,
+ "pos_y": -110.09375,
+ "pos_z": -98.5625,
+ "stations": "Irvin Beacon"
+ },
+ {
+ "name": "Norweilemil",
+ "pos_x": -49.28125,
+ "pos_y": -18.40625,
+ "pos_z": 87.09375,
+ "stations": "Szameit Works,Grant Survey,Smeaton's Progress"
+ },
+ {
+ "name": "Noti",
+ "pos_x": 72.15625,
+ "pos_y": 42.8125,
+ "pos_z": -31.4375,
+ "stations": "Weber Gateway,Dietz's Progress,Precourt City,Lazutkin Orbital,Heinlein Holdings"
+ },
+ {
+ "name": "Nott",
+ "pos_x": 36.53125,
+ "pos_y": 33.75,
+ "pos_z": -33.75,
+ "stations": "Maid Marian's Rest"
+ },
+ {
+ "name": "Notus",
+ "pos_x": -62.78125,
+ "pos_y": -19,
+ "pos_z": 4.90625,
+ "stations": "McDaniel Dock"
+ },
+ {
+ "name": "Novana",
+ "pos_x": -31.25,
+ "pos_y": 54.5625,
+ "pos_z": -109.90625,
+ "stations": "Behnken Terminal,Deere Settlement"
+ },
+ {
+ "name": "Novantait",
+ "pos_x": 85.71875,
+ "pos_y": -39.75,
+ "pos_z": 134.625,
+ "stations": "Cabot Prospect"
+ },
+ {
+ "name": "Novants",
+ "pos_x": -151.46875,
+ "pos_y": 7.3125,
+ "pos_z": 1.84375,
+ "stations": "Farkas City"
+ },
+ {
+ "name": "Novas",
+ "pos_x": 109.03125,
+ "pos_y": -0.25,
+ "pos_z": 71.84375,
+ "stations": "Porges Ring,McDonald City,Lowry City,Marques Station,Altshuller Dock,Isherwood Station,Moorcock Hub,Katzenstein Enterprise,Bloch Base,Chaviano Depot"
+ },
+ {
+ "name": "Novgoreng",
+ "pos_x": -104.5625,
+ "pos_y": -64.875,
+ "pos_z": 99.6875,
+ "stations": "Robinson Base"
+ },
+ {
+ "name": "Novgorod",
+ "pos_x": 76.46875,
+ "pos_y": 4.53125,
+ "pos_z": -130.875,
+ "stations": "Barton Dock,Fiennes Landing"
+ },
+ {
+ "name": "Novgorots",
+ "pos_x": 109.5625,
+ "pos_y": 58.53125,
+ "pos_z": -60.96875,
+ "stations": "Gamow Base,Kippax Refinery,Dobzhansky Terminal"
+ },
+ {
+ "name": "Nox",
+ "pos_x": 38.84375,
+ "pos_y": -17.78125,
+ "pos_z": -63.875,
+ "stations": "Berners-Lee Gateway,Anning Gateway,Mastracchio Enterprise,Schiltberger Keep"
+ },
+ {
+ "name": "NSV 3315",
+ "pos_x": 50.46875,
+ "pos_y": -4.8125,
+ "pos_z": -49.40625,
+ "stations": "Winne Station,Volk Plant"
+ },
+ {
+ "name": "Ntumusho",
+ "pos_x": 166.5625,
+ "pos_y": -194.4375,
+ "pos_z": 17.8125,
+ "stations": "Ryan Mines,Trimble Vision,Bauman's Claim"
+ },
+ {
+ "name": "Nu",
+ "pos_x": 71.375,
+ "pos_y": -114.5,
+ "pos_z": 15.09375,
+ "stations": "Syromyatnikov Horizons,Marius Orbital,Sy Base"
+ },
+ {
+ "name": "Nu Caeli",
+ "pos_x": 120.5625,
+ "pos_y": -108.6875,
+ "pos_z": -55.0625,
+ "stations": "Francis Station"
+ },
+ {
+ "name": "Nu Chamaelontis",
+ "pos_x": 161.4375,
+ "pos_y": -56.4375,
+ "pos_z": 68.875,
+ "stations": "Rosenberger Vision,Tusi Dock,Riazuddin Silo,Verrier Enterprise"
+ },
+ {
+ "name": "Nu Gong",
+ "pos_x": 57.1875,
+ "pos_y": -79.59375,
+ "pos_z": 154.71875,
+ "stations": "Calatrava Camp"
+ },
+ {
+ "name": "Nu Goryni",
+ "pos_x": 20.125,
+ "pos_y": 80.25,
+ "pos_z": -20.1875,
+ "stations": "Fraser Holdings,Tavernier Works,Hunt Vision,Alvares Barracks"
+ },
+ {
+ "name": "Nu Gu",
+ "pos_x": 81.53125,
+ "pos_y": 39.96875,
+ "pos_z": -115.59375,
+ "stations": "Bendell Dock,Ron Hubbard Colony,Pohl's Inheritance"
+ },
+ {
+ "name": "Nu Guambi",
+ "pos_x": -114.09375,
+ "pos_y": 7.25,
+ "pos_z": -47.625,
+ "stations": "Penn Barracks,Walker Ring,Ibold Terminal"
+ },
+ {
+ "name": "Nu Guana",
+ "pos_x": -96.375,
+ "pos_y": -138.34375,
+ "pos_z": -2.8125,
+ "stations": "Martin Survey"
+ },
+ {
+ "name": "Nu Guang",
+ "pos_x": -129,
+ "pos_y": -58.90625,
+ "pos_z": -32.0625,
+ "stations": "Herrington Gateway,Sadi Carnot Ring,Descartes Terminal,Vela Hub,Veach Gateway,Ampere Port,Rutherford Arsenal"
+ },
+ {
+ "name": "Nu Guei He",
+ "pos_x": -58.78125,
+ "pos_y": 29.25,
+ "pos_z": 159.4375,
+ "stations": "Locke Mines"
+ },
+ {
+ "name": "Nu Horologii",
+ "pos_x": 104.3125,
+ "pos_y": -125.1875,
+ "pos_z": 22.90625,
+ "stations": "Danjon Landing,Abbott Reach,Messerschmitt's Progress"
+ },
+ {
+ "name": "Nu Indi",
+ "pos_x": 48.3125,
+ "pos_y": -61.53125,
+ "pos_z": 51.4375,
+ "stations": "Northrop Enterprise,Shatalov Colony,Phillifent's Inheritance"
+ },
+ {
+ "name": "Nu Kop",
+ "pos_x": 132.09375,
+ "pos_y": -80.34375,
+ "pos_z": 33.75,
+ "stations": "Winthrop Landing,Baydukov Prospect,Polikarpov Horizons,Muramatsu Base"
+ },
+ {
+ "name": "Nu Kuan",
+ "pos_x": -161.5,
+ "pos_y": -23.4375,
+ "pos_z": -42.34375,
+ "stations": "Bacigalupi Holdings,Baraniecki Port,Hiroyuki Park,Cousin Prospect,Griffith Landing"
+ },
+ {
+ "name": "Nu Kuang",
+ "pos_x": 34.21875,
+ "pos_y": -94.59375,
+ "pos_z": -126.65625,
+ "stations": "Bean Station,Bobko Dock,Lobachevsky Base,Vesalius Vision,Morgan Keep"
+ },
+ {
+ "name": "Nu Kuni",
+ "pos_x": 28.625,
+ "pos_y": -97.0625,
+ "pos_z": 17.5625,
+ "stations": "Piazzi Hub,Moskowitz Prospect,van Rhijn City"
+ },
+ {
+ "name": "Nu Pictoris",
+ "pos_x": 140.9375,
+ "pos_y": -69.125,
+ "pos_z": -11.75,
+ "stations": "Fraknoi Platform,Adams Holdings,Alcock Prospect"
+ },
+ {
+ "name": "Nu Tauri",
+ "pos_x": 8.65625,
+ "pos_y": -62.78125,
+ "pos_z": -98.3125,
+ "stations": "Parmitano Terminal,Faraday Dock,Alcala Hub,Napier Barracks,Fujimori Horizons,Simbad's Refuge"
+ },
+ {
+ "name": "Nu Wana",
+ "pos_x": -60.4375,
+ "pos_y": -87.25,
+ "pos_z": -52.03125,
+ "stations": "Cori Colony,Shuttleworth Platform,Bamford Settlement,Becquerel Terminal,Vries Enterprise"
+ },
+ {
+ "name": "Nu Wanga",
+ "pos_x": -65.03125,
+ "pos_y": 49.34375,
+ "pos_z": -115.15625,
+ "stations": "Wilson City,Stirling Enterprise,Mondeh Dock,Bulleid Port,Babbage Enterprise,Rzeppa Holdings,Soukup Hub,Humboldt Enterprise,Snyder City,Burbank Orbital,Grimwood's Progress"
+ },
+ {
+ "name": "Nu-1 Columbae",
+ "pos_x": 90,
+ "pos_y": -58.65625,
+ "pos_z": -70.3125,
+ "stations": "Lloyd Terminal,Rond d'Alembert Ring,Stackpole Station,Moore Ring,Carlisle Ring,Teng Port"
+ },
+ {
+ "name": "Nu-1 Lupi",
+ "pos_x": 61.90625,
+ "pos_y": 14.65625,
+ "pos_z": 98.09375,
+ "stations": "Gernhardt Plant,Dupuy de Lome Horizons,Skripochka Hangar"
+ },
+ {
+ "name": "Nu-2 Canis Majoris",
+ "pos_x": 47.625,
+ "pos_y": -12.6875,
+ "pos_z": -41.5,
+ "stations": "Laveykin Settlement,Bolotov Vision"
+ },
+ {
+ "name": "Nu-2 Columbae",
+ "pos_x": 94.3125,
+ "pos_y": -61.125,
+ "pos_z": -71.28125,
+ "stations": "Pond's Folly,Al-Jazari's Progress,Zetford Settlement"
+ },
+ {
+ "name": "Nu-2 Lupi",
+ "pos_x": 25.78125,
+ "pos_y": 5.78125,
+ "pos_z": 40.4375,
+ "stations": "Pudwill Gorie Holdings,West Asylum,Gauss City"
+ },
+ {
+ "name": "Nuakea",
+ "pos_x": -19.6875,
+ "pos_y": 15.4375,
+ "pos_z": 47.0625,
+ "stations": "Admiral Thirsk,Oblivion,Stuart Hub,Scheutz Depot,Vizcaino Palace"
+ },
+ {
+ "name": "Nuen",
+ "pos_x": -141.25,
+ "pos_y": -59.53125,
+ "pos_z": 65.625,
+ "stations": "Stasheff Port,Sladek Ring,Minkowski Horizons,Flynn Works"
+ },
+ {
+ "name": "Nuenets",
+ "pos_x": 48,
+ "pos_y": 17.65625,
+ "pos_z": 28.3125,
+ "stations": "Laliberte Enterprise,Shriver Hub,Kadenyuk Palace,Ryazanski Dock,Betancourt Enterprise,Harbaugh Port,Forrester Dock"
+ },
+ {
+ "name": "Nuenoch",
+ "pos_x": -24.15625,
+ "pos_y": 16.34375,
+ "pos_z": 95.5625,
+ "stations": "Baudry Hub,Gibson Refinery,Maybury Penal colony,Sharma Prospect"
+ },
+ {
+ "name": "Nugua",
+ "pos_x": 170.0625,
+ "pos_y": 13.90625,
+ "pos_z": 68.6875,
+ "stations": "Kummer Vision,Kube-McDowell Gateway,Sladek Orbital,Barreiros Enterprise,Boulle Barracks"
+ },
+ {
+ "name": "Nuguynici",
+ "pos_x": -132.3125,
+ "pos_y": 44.40625,
+ "pos_z": -13.84375,
+ "stations": "Kirchoff Depot"
+ },
+ {
+ "name": "Nuit",
+ "pos_x": -100.8125,
+ "pos_y": -143.40625,
+ "pos_z": 35.875,
+ "stations": "Harvey Works,Leavitt Port,Bushkov Beacon"
+ },
+ {
+ "name": "Nuitae",
+ "pos_x": -145.65625,
+ "pos_y": 17.9375,
+ "pos_z": 59.1875,
+ "stations": "Fontenay Bastion,Arthur Dock"
+ },
+ {
+ "name": "Nuitati",
+ "pos_x": -36.6875,
+ "pos_y": 34.125,
+ "pos_z": -154.65625,
+ "stations": "Eisenstein Settlement,Andrews Port"
+ },
+ {
+ "name": "Nuite",
+ "pos_x": 44.875,
+ "pos_y": -1.53125,
+ "pos_z": -105.84375,
+ "stations": "Franklin Arsenal,Tanner Depot"
+ },
+ {
+ "name": "Nuites",
+ "pos_x": 53.25,
+ "pos_y": -41.6875,
+ "pos_z": -74.90625,
+ "stations": "McClintock City,Wilhelm Terminal,Simonyi Base,Conway Hangar"
+ },
+ {
+ "name": "Nuitu",
+ "pos_x": 0.25,
+ "pos_y": -22.65625,
+ "pos_z": -140.59375,
+ "stations": "Adams Enterprise,Borman Dock,Sharipov Terminal"
+ },
+ {
+ "name": "Nujema",
+ "pos_x": -82.46875,
+ "pos_y": -16.5625,
+ "pos_z": 104.03125,
+ "stations": "Heinlein Plant,McMahon Hub,Clauss Enterprise"
+ },
+ {
+ "name": "Nujemana",
+ "pos_x": -97.3125,
+ "pos_y": 99.03125,
+ "pos_z": -94.09375,
+ "stations": "Bagian Orbital"
+ },
+ {
+ "name": "Nukaitu",
+ "pos_x": 2.5,
+ "pos_y": 29.96875,
+ "pos_z": 133.03125,
+ "stations": "Barnwell Ring"
+ },
+ {
+ "name": "Nukam",
+ "pos_x": -0.9375,
+ "pos_y": 30.71875,
+ "pos_z": 65.3125,
+ "stations": "Swigert Holdings"
+ },
+ {
+ "name": "Nukama",
+ "pos_x": 128.96875,
+ "pos_y": -76.3125,
+ "pos_z": 39.46875,
+ "stations": "Bohrmann City,Hughes Station,Nijland Port,Jeans Hub,Gutierrez Orbital"
+ },
+ {
+ "name": "Nukamba",
+ "pos_x": -149.75,
+ "pos_y": 82.5,
+ "pos_z": 50.71875,
+ "stations": "Koontz Survey"
+ },
+ {
+ "name": "Nukunda",
+ "pos_x": -107.53125,
+ "pos_y": -49.03125,
+ "pos_z": 39.09375,
+ "stations": "Lee Dock,Stevens Base,Vardeman Point"
+ },
+ {
+ "name": "Nukups",
+ "pos_x": 106.9375,
+ "pos_y": -92.5,
+ "pos_z": -84.03125,
+ "stations": "Verne Terminal,Lie Gateway,Fidalgo Landing"
+ },
+ {
+ "name": "Nukuru",
+ "pos_x": -68,
+ "pos_y": 63.3125,
+ "pos_z": 138.3125,
+ "stations": "Haller Port,Eyharts Enterprise,Potagos Landing"
+ },
+ {
+ "name": "Nulili",
+ "pos_x": 7.625,
+ "pos_y": 116.59375,
+ "pos_z": 57,
+ "stations": "Singer Outpost"
+ },
+ {
+ "name": "Nulis",
+ "pos_x": -137.84375,
+ "pos_y": -13.875,
+ "pos_z": 27.15625,
+ "stations": "Baudin Colony,Carter Installation,Sakers Dock"
+ },
+ {
+ "name": "Nummitra",
+ "pos_x": 42.3125,
+ "pos_y": -178.53125,
+ "pos_z": 55.5,
+ "stations": "Hildebrandt Ring,Kirkwood Orbital,Courvoisier Vision,Mead Legacy"
+ },
+ {
+ "name": "Nun",
+ "pos_x": 100.65625,
+ "pos_y": -143.125,
+ "pos_z": 118.4375,
+ "stations": "Bharadwaj Port,Ziewe Hub,Young Penal colony,Vardeman Plant"
+ },
+ {
+ "name": "Nuna",
+ "pos_x": 149.0625,
+ "pos_y": -128.09375,
+ "pos_z": 58.0625,
+ "stations": "McKie Barracks,Gustav Sporer Horizons,Stephan Port"
+ },
+ {
+ "name": "Nunet",
+ "pos_x": 102.8125,
+ "pos_y": -3.03125,
+ "pos_z": 7,
+ "stations": "Gardner Hangar"
+ },
+ {
+ "name": "Nuneti",
+ "pos_x": -122.46875,
+ "pos_y": -44.28125,
+ "pos_z": -63.84375,
+ "stations": "Drake Landing"
+ },
+ {
+ "name": "Nungamici",
+ "pos_x": 28.4375,
+ "pos_y": -71.5625,
+ "pos_z": 90.65625,
+ "stations": "Chang-Diaz Terminal,Thiele Port,Galvani Hub,Matteucci Station,Roosa Hub,Lazutkin Holdings"
+ },
+ {
+ "name": "Nungar",
+ "pos_x": 97.59375,
+ "pos_y": -16.09375,
+ "pos_z": 7.4375,
+ "stations": "Weitz Vision,Al-Farabi Mines,Binet Enterprise,Ashton Hub"
+ },
+ {
+ "name": "Nunggul",
+ "pos_x": 80.53125,
+ "pos_y": 155.5625,
+ "pos_z": -32.65625,
+ "stations": "Lenthall Hub,Watts Beacon,McAllaster Oasis"
+ },
+ {
+ "name": "Nungk",
+ "pos_x": 136.90625,
+ "pos_y": -65.4375,
+ "pos_z": 129.34375,
+ "stations": "Arai Orbital,Ilyushin Vision,MacGill Orbital,Cartier Beacon"
+ },
+ {
+ "name": "Nungnges",
+ "pos_x": 179.46875,
+ "pos_y": -125.28125,
+ "pos_z": -1.8125,
+ "stations": "Resnik Dock,Hirn Station,Qian Terminal"
+ },
+ {
+ "name": "Nunu",
+ "pos_x": 11.25,
+ "pos_y": -111.40625,
+ "pos_z": -140.15625,
+ "stations": "Ockels Works,Delany Legacy,al-Haytham Terminal"
+ },
+ {
+ "name": "Nununni",
+ "pos_x": 110.3125,
+ "pos_y": 9.75,
+ "pos_z": 45.59375,
+ "stations": "Lawrence Orbital,Ericsson Station"
+ },
+ {
+ "name": "Nunuri",
+ "pos_x": 136.4375,
+ "pos_y": 46.40625,
+ "pos_z": -43.25,
+ "stations": "Watson Colony"
+ },
+ {
+ "name": "Nunus",
+ "pos_x": 82.40625,
+ "pos_y": -203.4375,
+ "pos_z": 44.125,
+ "stations": "Antoniadi Port,Peebles Gateway,Wenzel Terminal,Sternbach Vision,Froud Ring"
+ },
+ {
+ "name": "Nurundere",
+ "pos_x": -86.34375,
+ "pos_y": 5.71875,
+ "pos_z": -82.96875,
+ "stations": "Gubarev Port,Gagnan Orbital"
+ },
+ {
+ "name": "Nurundiji",
+ "pos_x": 70.125,
+ "pos_y": -180.09375,
+ "pos_z": 88.21875,
+ "stations": "Pimi Dock,Aitken Stop"
+ },
+ {
+ "name": "Nurungu",
+ "pos_x": 105.4375,
+ "pos_y": 66.90625,
+ "pos_z": -79.65625,
+ "stations": "Paulo da Gama Point"
+ },
+ {
+ "name": "Nusakan",
+ "pos_x": -46.40625,
+ "pos_y": 93.5625,
+ "pos_z": 45.75,
+ "stations": "Bacon City,Nasmyth Port,Bergerac Base"
+ },
+ {
+ "name": "Nut",
+ "pos_x": 57.90625,
+ "pos_y": -129.5,
+ "pos_z": -67.9375,
+ "stations": "Babcock Gateway,Heiles Dock,Shavyrin Station,Berezin Lab,Napier Park"
+ },
+ {
+ "name": "Nuwach",
+ "pos_x": -48.46875,
+ "pos_y": -12.96875,
+ "pos_z": 183,
+ "stations": "Semeonis Hub,Ferguson Hub,Sagan Ring,Gotlieb Lab,Brothers Bastion"
+ },
+ {
+ "name": "Nuxalis",
+ "pos_x": 53.96875,
+ "pos_y": 97.96875,
+ "pos_z": -37.84375,
+ "stations": "Ito Outpost,Blodgett Platform"
+ },
+ {
+ "name": "Nuxalkuq",
+ "pos_x": 149.53125,
+ "pos_y": -182.9375,
+ "pos_z": 74,
+ "stations": "Naubakht Terminal,Beg Port"
+ },
+ {
+ "name": "Nyal",
+ "pos_x": -18.28125,
+ "pos_y": -11.65625,
+ "pos_z": -104.40625,
+ "stations": "Chadwick Colony,Smith Vision,MacLean's Inheritance"
+ },
+ {
+ "name": "Nyala",
+ "pos_x": -45.8125,
+ "pos_y": -2.625,
+ "pos_z": -138.5,
+ "stations": "Wilkes' Progress,Malenchenko's Inheritance,Olivas Terminal,Becquerel Terminal,Creighton Terminal"
+ },
+ {
+ "name": "Nyalalandi",
+ "pos_x": -32.625,
+ "pos_y": -30.03125,
+ "pos_z": 160.5625,
+ "stations": "Williamson Holdings"
+ },
+ {
+ "name": "Nyalayan",
+ "pos_x": 29.71875,
+ "pos_y": -172.71875,
+ "pos_z": -6.46875,
+ "stations": "Peters Hub,Pilcher Port,Folland Port,Sutter Ring,Lyne Vision,Arfstrom Legacy,Gray Vision,Bouwens Vision,Rubin Orbital"
+ },
+ {
+ "name": "Nyaliep",
+ "pos_x": -49.6875,
+ "pos_y": -20.84375,
+ "pos_z": 168.78125,
+ "stations": "Markov Prospect"
+ },
+ {
+ "name": "Nyalo",
+ "pos_x": -119.8125,
+ "pos_y": -55.3125,
+ "pos_z": -42.96875,
+ "stations": "Forsskal Landing,Chapman Vision,Burke Terminal"
+ },
+ {
+ "name": "Nyamad",
+ "pos_x": -141.8125,
+ "pos_y": 6.65625,
+ "pos_z": -35.75,
+ "stations": "Lawhead Mines"
+ },
+ {
+ "name": "Nyamari",
+ "pos_x": -78.125,
+ "pos_y": 95.875,
+ "pos_z": 57.28125,
+ "stations": "Narita Legacy,Amis Survey"
+ },
+ {
+ "name": "Nyament",
+ "pos_x": 101.90625,
+ "pos_y": -246.8125,
+ "pos_z": 129.34375,
+ "stations": "Sorayama Orbital,Lindblad Landing,Luyten Orbital,Herbert Beacon,Abe Relay"
+ },
+ {
+ "name": "Nyaminawe",
+ "pos_x": -1.34375,
+ "pos_y": -83.125,
+ "pos_z": 86.46875,
+ "stations": "Brosnatch Installation,Burnham Station"
+ },
+ {
+ "name": "Nyamwere",
+ "pos_x": 103.90625,
+ "pos_y": -85.5,
+ "pos_z": 130.15625,
+ "stations": "Roth City,Dolgov Works,Hell Station,Heinkel Terminal,Galle Legacy"
+ },
+ {
+ "name": "Nyamwezi",
+ "pos_x": 152.3125,
+ "pos_y": 57,
+ "pos_z": 15.125,
+ "stations": "Karl Diesel Depot"
+ },
+ {
+ "name": "Nyan",
+ "pos_x": 20.0625,
+ "pos_y": -180.53125,
+ "pos_z": -23.09375,
+ "stations": "Ledyard Survey,Oshima's Claim,Sugie Works"
+ },
+ {
+ "name": "Nyanga",
+ "pos_x": 88.1875,
+ "pos_y": -8.875,
+ "pos_z": -58.46875,
+ "stations": "Sarmiento de Gamboa Prospect"
+ },
+ {
+ "name": "Nyani",
+ "pos_x": 154.71875,
+ "pos_y": 10.03125,
+ "pos_z": -99.53125,
+ "stations": "Thompson Stop"
+ },
+ {
+ "name": "Nyania",
+ "pos_x": -40.84375,
+ "pos_y": 30.96875,
+ "pos_z": 162.0625,
+ "stations": "Harrison Vision,Reiter Vision"
+ },
+ {
+ "name": "Nyanktona",
+ "pos_x": 69.0625,
+ "pos_y": 16.03125,
+ "pos_z": 61.15625,
+ "stations": "Midgeley Hub,Acaba Horizons,Herreshoff Installation,MacKellar Dock"
+ },
+ {
+ "name": "Nyanmil",
+ "pos_x": 15.5625,
+ "pos_y": 63.9375,
+ "pos_z": 85.46875,
+ "stations": "Walheim Enterprise,Furrer Ring,Aldrin Terminal,Gagnan Holdings"
+ },
+ {
+ "name": "Nyanomam",
+ "pos_x": 36.71875,
+ "pos_y": -83.6875,
+ "pos_z": 146.78125,
+ "stations": "Moran Landing,Reed Dock,Greenleaf Base"
+ },
+ {
+ "name": "Nyanook",
+ "pos_x": 78.40625,
+ "pos_y": -189.125,
+ "pos_z": 121.0625,
+ "stations": "Lynden-Bell Platform,Arnold Prospect,Marques Base"
+ },
+ {
+ "name": "Nyeajaae VU-Y a27-9",
+ "pos_x": -3374,
+ "pos_y": -42.375,
+ "pos_z": 6907.96875,
+ "stations": "Mjolnir's Wrath"
+ },
+ {
+ "name": "Nyikamana",
+ "pos_x": 95.34375,
+ "pos_y": 22.375,
+ "pos_z": -47.53125,
+ "stations": "Hottot Holdings,Mourelle Prospect,Daley Prospect"
+ },
+ {
+ "name": "Nyikan",
+ "pos_x": 19.875,
+ "pos_y": -125.875,
+ "pos_z": 124.53125,
+ "stations": "Brust Laboratory,Flammarion Vision"
+ },
+ {
+ "name": "Nyikara",
+ "pos_x": -9,
+ "pos_y": -182.3125,
+ "pos_z": 77.6875,
+ "stations": "Mizuno Terminal,Florine Port,Al-Khujandi Works"
+ },
+ {
+ "name": "Nyikari",
+ "pos_x": 8.65625,
+ "pos_y": -7.1875,
+ "pos_z": 137.6875,
+ "stations": "Foale Vision"
+ },
+ {
+ "name": "Nyikas",
+ "pos_x": 44.34375,
+ "pos_y": -171.84375,
+ "pos_z": -63.125,
+ "stations": "Mikoyan Vision"
+ },
+ {
+ "name": "Nyikates",
+ "pos_x": -113.28125,
+ "pos_y": -44.59375,
+ "pos_z": -4.09375,
+ "stations": "Gibson Holdings,Boe Colony"
+ },
+ {
+ "name": "Nyiko Oh",
+ "pos_x": -124.65625,
+ "pos_y": 77.8125,
+ "pos_z": -55.3125,
+ "stations": "Ponce de Leon Settlement,Le Guin Holdings"
+ },
+ {
+ "name": "Nyikoku",
+ "pos_x": -182.59375,
+ "pos_y": 28.0625,
+ "pos_z": -25.3125,
+ "stations": "Lethem Platform"
+ },
+ {
+ "name": "Nyokojai",
+ "pos_x": -82.90625,
+ "pos_y": -10.75,
+ "pos_z": 35.1875,
+ "stations": "Cardano Terminal,Duckworth Prospect,Fernandes Hub"
+ },
+ {
+ "name": "Nyokomici",
+ "pos_x": -115.15625,
+ "pos_y": 19.21875,
+ "pos_z": 103.6875,
+ "stations": "Rolland Ring"
+ },
+ {
+ "name": "Nyokot",
+ "pos_x": -54.6875,
+ "pos_y": -2.25,
+ "pos_z": -181,
+ "stations": "Lem Dock,Piserchia Enterprise,Hamilton Vision"
+ },
+ {
+ "name": "Nyora",
+ "pos_x": -159.5,
+ "pos_y": -4.09375,
+ "pos_z": 0.84375,
+ "stations": "Pasteur Port,Walters' Claim,Cabrera Dock,Watson Dock"
+ },
+ {
+ "name": "Nyore",
+ "pos_x": -127.5625,
+ "pos_y": 109.0625,
+ "pos_z": 1.625,
+ "stations": "Brady Beacon,Marley Works"
+ },
+ {
+ "name": "Nyorensei",
+ "pos_x": -104.125,
+ "pos_y": 79.8125,
+ "pos_z": -65.625,
+ "stations": "Rangarajan Dock"
+ },
+ {
+ "name": "Nyorenses",
+ "pos_x": -141.0625,
+ "pos_y": -2.65625,
+ "pos_z": 56.375,
+ "stations": "Dalmas Mines,Stephenson Penal colony"
+ },
+ {
+ "name": "Nyoro",
+ "pos_x": 136.125,
+ "pos_y": -75.65625,
+ "pos_z": -39.21875,
+ "stations": "Baracchi Hub,Milne Depot"
+ },
+ {
+ "name": "Nyororor",
+ "pos_x": 80.59375,
+ "pos_y": -168.3125,
+ "pos_z": -60.1875,
+ "stations": "Biermann Dock,Baade Laboratory,Harvia Station,Okuni Relay"
+ },
+ {
+ "name": "Nyoru",
+ "pos_x": 8.59375,
+ "pos_y": -63.9375,
+ "pos_z": 80.6875,
+ "stations": "Bombelli Port,Evans Barracks,Foster Relay"
+ },
+ {
+ "name": "Nysa",
+ "pos_x": 88.96875,
+ "pos_y": -114.5,
+ "pos_z": -6.71875,
+ "stations": "Herreshoff Barracks,Olahus Installation,Dolgov Plant,Arend Station"
+ },
+ {
+ "name": "Nyx",
+ "pos_x": -29.4375,
+ "pos_y": -68.78125,
+ "pos_z": -11.5,
+ "stations": "Shkaplerov Hub,Viktorenko Port,Ryumin Orbital,Vinogradov Orbital,Wolf Dock,Howe Station,Gemar Dock,Chiao City,Montrose Hub,Boodt Prospect,Ali City,Watt Base,Adams Holdings,Ford Installation,Hodgson Mine"
+ },
+ {
+ "name": "Nzam Caihe",
+ "pos_x": 49.3125,
+ "pos_y": -153.65625,
+ "pos_z": -88.09375,
+ "stations": "Artzybasheff Landing"
+ },
+ {
+ "name": "Nzam Cakua",
+ "pos_x": -90.65625,
+ "pos_y": 8.5,
+ "pos_z": -40.625,
+ "stations": "Grigson Terminal,Young Station"
+ },
+ {
+ "name": "Nzam Cimi",
+ "pos_x": -132.90625,
+ "pos_y": 15.78125,
+ "pos_z": 39.4375,
+ "stations": "Wrangell Orbital,Newcomen Dock,Galilei Gateway,Vardeman Works"
+ },
+ {
+ "name": "Nzambassee",
+ "pos_x": -1.03125,
+ "pos_y": 33.0625,
+ "pos_z": -19.96875,
+ "stations": "Broderick Orbital,Montrose Gateway,Arnarson Ring,Manning Orbital"
+ },
+ {
+ "name": "Nzamnet",
+ "pos_x": 83.53125,
+ "pos_y": -87.5,
+ "pos_z": 15.1875,
+ "stations": "Kippax Keep,Draper Dock,Jones Vision"
+ },
+ {
+ "name": "Nzamnite",
+ "pos_x": -5.625,
+ "pos_y": -4.9375,
+ "pos_z": 100.78125,
+ "stations": "Satcher Hub,Kelly Station,Low Bastion,Magnus Orbital,Serre Port,Wallin Survey"
+ },
+ {
+ "name": "Oanoa",
+ "pos_x": -1.15625,
+ "pos_y": 39.21875,
+ "pos_z": 158.40625,
+ "stations": "Low Survey"
+ },
+ {
+ "name": "Obaluaye",
+ "pos_x": 67.09375,
+ "pos_y": -95.1875,
+ "pos_z": 93.84375,
+ "stations": "Lyne Settlement"
+ },
+ {
+ "name": "Obamba",
+ "pos_x": -104.46875,
+ "pos_y": -14.40625,
+ "pos_z": 90.34375,
+ "stations": "Serre's Pride,Bordage Sanctuary"
+ },
+ {
+ "name": "Obambivas",
+ "pos_x": -59.9375,
+ "pos_y": 7.375,
+ "pos_z": 56.8125,
+ "stations": "Kennan Orbital,Galouye Terminal,Clebsch Terminal,Hasse Terminal,Kuipers Landing,RenenBellot Installation"
+ },
+ {
+ "name": "Obambojas",
+ "pos_x": -30.375,
+ "pos_y": -10.09375,
+ "pos_z": 114.3125,
+ "stations": "Wheeler Hub,Williams Depot,Melvill Gateway,Meitner Hub,Bates Base"
+ },
+ {
+ "name": "Obambori",
+ "pos_x": -6.46875,
+ "pos_y": -22.8125,
+ "pos_z": 160,
+ "stations": "Norgay Hub,Jeury Silo"
+ },
+ {
+ "name": "Obamboro",
+ "pos_x": -126.09375,
+ "pos_y": -31.78125,
+ "pos_z": -82.53125,
+ "stations": "Steele Dock,Tudela Works,Marlowe Beacon,Hand Settlement"
+ },
+ {
+ "name": "Obamulo",
+ "pos_x": 150.8125,
+ "pos_y": -175.65625,
+ "pos_z": 76.15625,
+ "stations": "Ohain Orbital,Elbakyan Terminal"
+ },
+ {
+ "name": "Obamumbo",
+ "pos_x": -9.03125,
+ "pos_y": -78.59375,
+ "pos_z": -152.90625,
+ "stations": "Ivanchenkov Hub,Yegorov Gateway,Cormack Beacon,Trinh Enterprise,Alvarado Beacon"
+ },
+ {
+ "name": "Obassi Osaw",
+ "pos_x": -53.96875,
+ "pos_y": -144.53125,
+ "pos_z": -96.9375,
+ "stations": "Teller Horizons,Feustel Dock"
+ },
+ {
+ "name": "Obastaragea",
+ "pos_x": 114.59375,
+ "pos_y": -209.59375,
+ "pos_z": 19.53125,
+ "stations": "Gabrielli Mines,Kemurdzhian Survey,Ozanne Prospect"
+ },
+ {
+ "name": "Obastini",
+ "pos_x": 81.40625,
+ "pos_y": 46,
+ "pos_z": 101.0625,
+ "stations": "Vittori Settlement,Jahn Dock,Mukai Refinery"
+ },
+ {
+ "name": "Obates",
+ "pos_x": 62.21875,
+ "pos_y": 94.96875,
+ "pos_z": 108.34375,
+ "stations": "Lowry Terminal,Henson Landing,Reed Colony,Viete Gateway"
+ },
+ {
+ "name": "Obato",
+ "pos_x": -23.9375,
+ "pos_y": -42.0625,
+ "pos_z": -88.53125,
+ "stations": "Cseszneky Ring,Stanley Holdings,Fossey Terminal,Zillig Vision,Dzhanibekov Terminal"
+ },
+ {
+ "name": "Obe",
+ "pos_x": -59.09375,
+ "pos_y": -46.25,
+ "pos_z": 66.03125,
+ "stations": "Euler Base"
+ },
+ {
+ "name": "Obotra",
+ "pos_x": -5.09375,
+ "pos_y": -23.625,
+ "pos_z": -152.3125,
+ "stations": "Needham Depot"
+ },
+ {
+ "name": "Obotrima",
+ "pos_x": 24.1875,
+ "pos_y": -67.53125,
+ "pos_z": -0.6875,
+ "stations": "Fujimori Keep,Davis Dock,Thoreau Orbital"
+ },
+ {
+ "name": "Obotrimi",
+ "pos_x": -66.75,
+ "pos_y": -58.875,
+ "pos_z": 28.40625,
+ "stations": "Buffett City,Grabe Orbital"
+ },
+ {
+ "name": "Obotrimpox",
+ "pos_x": 137.46875,
+ "pos_y": 20.5625,
+ "pos_z": -2.875,
+ "stations": "Donaldson Hub,Zewail Relay,Leslie Terminal"
+ },
+ {
+ "name": "Ocaina",
+ "pos_x": -64,
+ "pos_y": -44.65625,
+ "pos_z": 95.5625,
+ "stations": "Vinci Ring,Narvaez Silo"
+ },
+ {
+ "name": "Ocainawaka",
+ "pos_x": -49.90625,
+ "pos_y": -149.625,
+ "pos_z": -56.59375,
+ "stations": "Qureshi Depot"
+ },
+ {
+ "name": "Ocainn",
+ "pos_x": 0.3125,
+ "pos_y": -124.34375,
+ "pos_z": -48.15625,
+ "stations": "Walz Plant,Hoften Plant"
+ },
+ {
+ "name": "Ocairi",
+ "pos_x": 116.34375,
+ "pos_y": -86.1875,
+ "pos_z": 31.78125,
+ "stations": "Marius Dock,Schroter Beacon,Reeves City,Maitz Ring,McKean Dock,Bayer Orbital,Lunney Beacon,Mikoyan Gateway,Metcalf Station"
+ },
+ {
+ "name": "Ocassi",
+ "pos_x": 29.15625,
+ "pos_y": 112.90625,
+ "pos_z": -127.125,
+ "stations": "Schrodinger Base"
+ },
+ {
+ "name": "Ocelete",
+ "pos_x": -4.8125,
+ "pos_y": 49.28125,
+ "pos_z": -91.53125,
+ "stations": "Lee Barracks,Lysenko Dock"
+ },
+ {
+ "name": "Oceliu Anat",
+ "pos_x": -149,
+ "pos_y": -34.78125,
+ "pos_z": 56.375,
+ "stations": "Newman Orbital,Metcalf Enterprise,Haber Base"
+ },
+ {
+ "name": "Ocelliep",
+ "pos_x": 123.40625,
+ "pos_y": -86.53125,
+ "pos_z": -101.40625,
+ "stations": "Husband Station,Jung Vision"
+ },
+ {
+ "name": "Ochoeng",
+ "pos_x": -139.0625,
+ "pos_y": -2.3125,
+ "pos_z": -6.65625,
+ "stations": "Gotlieb Port,Roddenberry Gateway,Kube-McDowell Dock,Bisson Dock,Cixin Dock,Alten's Inheritance,Vance City,Alexandria Prospect,Perga Station"
+ },
+ {
+ "name": "Ochosag",
+ "pos_x": 115.125,
+ "pos_y": -21.84375,
+ "pos_z": -94.625,
+ "stations": "Babcock Horizons,Borman Prospect,Shaikh Point"
+ },
+ {
+ "name": "Ochoshei",
+ "pos_x": 110.96875,
+ "pos_y": 4.6875,
+ "pos_z": 68.78125,
+ "stations": "Mitchell Beacon,Arthur Reach"
+ },
+ {
+ "name": "Ochosi",
+ "pos_x": 27.15625,
+ "pos_y": 153.03125,
+ "pos_z": 68.65625,
+ "stations": "Perry's Folly,Sheckley Holdings"
+ },
+ {
+ "name": "Ocsho",
+ "pos_x": -51,
+ "pos_y": -18.75,
+ "pos_z": 94.71875,
+ "stations": "Stein Plant"
+ },
+ {
+ "name": "Ocshodhis",
+ "pos_x": -132.90625,
+ "pos_y": -25,
+ "pos_z": -60.84375,
+ "stations": "Baxter's Inheritance,Lloyd Dock"
+ },
+ {
+ "name": "Ocshongzi",
+ "pos_x": 129.375,
+ "pos_y": -12.28125,
+ "pos_z": -86.6875,
+ "stations": "Bischoff Vision,Belyanin Mines,Garrett Refinery"
+ },
+ {
+ "name": "Ocshooit",
+ "pos_x": 19.0625,
+ "pos_y": 139.40625,
+ "pos_z": -3.3125,
+ "stations": "Antonio de Andrade Settlement,Silva Port,Litke Plant,Parry Terminal,Makeev Gateway,Fife Vision"
+ },
+ {
+ "name": "Odinja",
+ "pos_x": 156.1875,
+ "pos_y": -2.96875,
+ "pos_z": 20.40625,
+ "stations": "LeConte Ring,Kingsmill Base,Hoffman Point"
+ },
+ {
+ "name": "Odins",
+ "pos_x": 99.53125,
+ "pos_y": -124.8125,
+ "pos_z": -24.5,
+ "stations": "Roche Orbital,Common City,Zamyatin Silo,Puiseux Port,Kiernan Installation"
+ },
+ {
+ "name": "Odomazotz",
+ "pos_x": 72.4375,
+ "pos_y": -9.53125,
+ "pos_z": 67.34375,
+ "stations": "Lloyd Wright Ring,Cummings Depot,Rosenberger Dock,Haldeman II Holdings,Molyneux Hub"
+ },
+ {
+ "name": "Odudu",
+ "pos_x": 13.6875,
+ "pos_y": 0.1875,
+ "pos_z": -174.59375,
+ "stations": "Scobee Dock"
+ },
+ {
+ "name": "Odudungu",
+ "pos_x": -16.75,
+ "pos_y": 23.4375,
+ "pos_z": -174.40625,
+ "stations": "Eddington Orbital,Slonczewski Arsenal"
+ },
+ {
+ "name": "Oduduni",
+ "pos_x": -172.1875,
+ "pos_y": 35.1875,
+ "pos_z": 26.25,
+ "stations": "Napier Landing,Brackett Settlement"
+ },
+ {
+ "name": "Odudunn",
+ "pos_x": 56.59375,
+ "pos_y": 98.5,
+ "pos_z": -80.6875,
+ "stations": "Tapinas Stop,Kraepelin Mines"
+ },
+ {
+ "name": "Oduduro",
+ "pos_x": -7.90625,
+ "pos_y": -23.375,
+ "pos_z": -31.625,
+ "stations": "Bao Prospect,Conrad Hub,McDivitt Dock,Deluc Arsenal,Dalton Gateway,Hawking Terminal,Heng Dock,Macomb Palace,Liebig Hub,Kraepelin Hub,Whitney Station,Thomas Vision,Dobrovolski Terminal,Drake Beacon,Halsell Laboratory"
+ },
+ {
+ "name": "Odudusekui",
+ "pos_x": 1.65625,
+ "pos_y": -207.96875,
+ "pos_z": 79.0625,
+ "stations": "Hunt Works,Waldeck Enterprise,Zarnecki Dock,Dornier Prospect"
+ },
+ {
+ "name": "Oduduseri",
+ "pos_x": 122.4375,
+ "pos_y": -9.25,
+ "pos_z": 39.09375,
+ "stations": "Fisher Enterprise"
+ },
+ {
+ "name": "Oduwaid Fo",
+ "pos_x": -58.03125,
+ "pos_y": 111.78125,
+ "pos_z": -72.25,
+ "stations": "Yano Terminal,Remek Base,Euthymenes Penal colony"
+ },
+ {
+ "name": "Oduwonggani",
+ "pos_x": 105.6875,
+ "pos_y": 127.09375,
+ "pos_z": 53.28125,
+ "stations": "Harrison Station"
+ },
+ {
+ "name": "Oeng",
+ "pos_x": -43.40625,
+ "pos_y": -120.6875,
+ "pos_z": 124.5625,
+ "stations": "Evans Works"
+ },
+ {
+ "name": "Oeng Bosat",
+ "pos_x": 40.15625,
+ "pos_y": -120.8125,
+ "pos_z": -117.65625,
+ "stations": "Fadlan Relay,Stanley Plant"
+ },
+ {
+ "name": "Oeng Ku",
+ "pos_x": 129.34375,
+ "pos_y": -204.46875,
+ "pos_z": 58.84375,
+ "stations": "Ortiz Moreno Silo,Addams Beacon"
+ },
+ {
+ "name": "Oeng Zhaob",
+ "pos_x": 56.34375,
+ "pos_y": -37.875,
+ "pos_z": 16.28125,
+ "stations": "Drummond Legacy,Nachtigal Outpost"
+ },
+ {
+ "name": "Ofan",
+ "pos_x": 45.75,
+ "pos_y": -16.78125,
+ "pos_z": 86.78125,
+ "stations": "Goeschke Dock,Polansky Prospect,Popovich Orbital,Barreiros Silo,Piaget Settlement"
+ },
+ {
+ "name": "Ofaye Aibe",
+ "pos_x": 69.3125,
+ "pos_y": -77,
+ "pos_z": -82,
+ "stations": "Maire's Claim,Pirsan Relay,Libby Relay"
+ },
+ {
+ "name": "Ofayeka",
+ "pos_x": 151.28125,
+ "pos_y": -141.21875,
+ "pos_z": -15.65625,
+ "stations": "Meech City,Maine Silo"
+ },
+ {
+ "name": "Ogdoad",
+ "pos_x": 139.90625,
+ "pos_y": -204.375,
+ "pos_z": -36.875,
+ "stations": "Roskam Enterprise,Kingsbury Survey"
+ },
+ {
+ "name": "Oghmangua",
+ "pos_x": -50.375,
+ "pos_y": -90.46875,
+ "pos_z": 99.75,
+ "stations": "Bushnell Retreat,Lindsey Oasis"
+ },
+ {
+ "name": "Oglan",
+ "pos_x": -25.75,
+ "pos_y": -165.3125,
+ "pos_z": 29.09375,
+ "stations": "Takamizawa Landing"
+ },
+ {
+ "name": "Ogma",
+ "pos_x": 90.8125,
+ "pos_y": 79.46875,
+ "pos_z": -5.59375,
+ "stations": "Eyharts Platform"
+ },
+ {
+ "name": "Ogmana",
+ "pos_x": 129.0625,
+ "pos_y": -135.03125,
+ "pos_z": -71.65625,
+ "stations": "Araki Dock"
+ },
+ {
+ "name": "Ogmar",
+ "pos_x": -9534,
+ "pos_y": -905.28125,
+ "pos_z": 19802.03125,
+ "stations": "Dervish Platform,Whirling Station"
+ },
+ {
+ "name": "Ogminegalku",
+ "pos_x": -127.375,
+ "pos_y": 3.125,
+ "pos_z": -97.78125,
+ "stations": "Timofeyevich's Claim,Bruce Settlement"
+ },
+ {
+ "name": "Ogminiquit",
+ "pos_x": 145.75,
+ "pos_y": -20.09375,
+ "pos_z": -102.375,
+ "stations": "Otto Station,Ham Ring,Sarmiento de Gamboa Vision,Blenkinsop Gateway,Menezes Enterprise"
+ },
+ {
+ "name": "Ogmiusitou",
+ "pos_x": -119.1875,
+ "pos_y": -82.59375,
+ "pos_z": 8.84375,
+ "stations": "Lyakhov Orbital,Seega Colony,Hunziker Installation,Kafka Reach,Sargent Exchange"
+ },
+ {
+ "name": "Ogmiutana",
+ "pos_x": 58.6875,
+ "pos_y": -22.96875,
+ "pos_z": -159.65625,
+ "stations": "Hughes-Fulford Platform"
+ },
+ {
+ "name": "Ogonda",
+ "pos_x": 199.96875,
+ "pos_y": -109.71875,
+ "pos_z": 34.4375,
+ "stations": "Somayaji Vision,Keeler Platform,Abell Settlement,Tshang Refinery,Finch Holdings"
+ },
+ {
+ "name": "Ogondage",
+ "pos_x": 80.21875,
+ "pos_y": 64.96875,
+ "pos_z": -36.21875,
+ "stations": "Thompson Station,Shalatula Dock,Henson Terminal,Schiltberger Dock,Barr Dock,Young Town,MacCurdy Port,Blish Hub,Tall Reach"
+ },
+ {
+ "name": "Ogones",
+ "pos_x": 81.8125,
+ "pos_y": -242.65625,
+ "pos_z": -4.84375,
+ "stations": "Heiles Reach"
+ },
+ {
+ "name": "Ogoni",
+ "pos_x": 63,
+ "pos_y": -193.75,
+ "pos_z": 14,
+ "stations": "Phillips Beacon,Allen Settlement"
+ },
+ {
+ "name": "Ogowei",
+ "pos_x": 69.78125,
+ "pos_y": -187.71875,
+ "pos_z": 87.21875,
+ "stations": "Beriev Landing,Farghani's Claim,Serrao Point"
+ },
+ {
+ "name": "Ogowen",
+ "pos_x": -91.15625,
+ "pos_y": -97.71875,
+ "pos_z": -48.8125,
+ "stations": "Bosch Settlement,Arrhenius Mines"
+ },
+ {
+ "name": "Ogowenae",
+ "pos_x": -38.96875,
+ "pos_y": 116.96875,
+ "pos_z": -44.28125,
+ "stations": "Dann Ring,Vancouver Relay,Lerner Prospect"
+ },
+ {
+ "name": "Ogowendes",
+ "pos_x": 55.5,
+ "pos_y": -63.5625,
+ "pos_z": 73.125,
+ "stations": "Hayashi Installation,Patry Settlement,Werber Relay"
+ },
+ {
+ "name": "Ogowenk",
+ "pos_x": -70.375,
+ "pos_y": 31.71875,
+ "pos_z": -76.8125,
+ "stations": "Steinmuller Outpost,Paez's Progress"
+ },
+ {
+ "name": "Ogunda",
+ "pos_x": 72.03125,
+ "pos_y": -16.78125,
+ "pos_z": -104.53125,
+ "stations": "Bridges Station,Nikitin's Folly,Swainson Orbital,Lynn Forum"
+ },
+ {
+ "name": "Ogungurugh",
+ "pos_x": 121.125,
+ "pos_y": -131.5625,
+ "pos_z": 125,
+ "stations": "Kerimov Stop"
+ },
+ {
+ "name": "Oguninksmii",
+ "pos_x": 80,
+ "pos_y": 47.84375,
+ "pos_z": 53.34375,
+ "stations": "Anderson Platform,Carlisle Dock,Nagel Laboratory"
+ },
+ {
+ "name": "Ogunnr",
+ "pos_x": 40.6875,
+ "pos_y": -41.21875,
+ "pos_z": -84.9375,
+ "stations": "Solovyev Ring"
+ },
+ {
+ "name": "Ogunnus",
+ "pos_x": 137.21875,
+ "pos_y": 83.25,
+ "pos_z": -5.28125,
+ "stations": "Nicollier's Claim,Evans Platform"
+ },
+ {
+ "name": "Oho Bajo",
+ "pos_x": -27.5625,
+ "pos_y": -6.3125,
+ "pos_z": 87.59375,
+ "stations": "Herrington Colony,Gardner Survey,Coke Landing"
+ },
+ {
+ "name": "Oicoan",
+ "pos_x": -136.125,
+ "pos_y": -43.78125,
+ "pos_z": 73.1875,
+ "stations": "Gromov Camp"
+ },
+ {
+ "name": "Oicolmas",
+ "pos_x": -84.4375,
+ "pos_y": -82.5,
+ "pos_z": -38.625,
+ "stations": "Lebesgue Prospect,Rey Point"
+ },
+ {
+ "name": "Oicorio",
+ "pos_x": 178.96875,
+ "pos_y": -180.15625,
+ "pos_z": 47.875,
+ "stations": "Wilson Port"
+ },
+ {
+ "name": "Oicornga",
+ "pos_x": 136.09375,
+ "pos_y": -206.65625,
+ "pos_z": -3.46875,
+ "stations": "Takahashi Station,Gorey Survey,Beebe Survey,Fawcett Horizons"
+ },
+ {
+ "name": "Oisi",
+ "pos_x": -44.8125,
+ "pos_y": 19.34375,
+ "pos_z": -102.375,
+ "stations": "Alvares Ring,Dozois Base,Sheckley Station"
+ },
+ {
+ "name": "Oitbi",
+ "pos_x": -44.09375,
+ "pos_y": -74.1875,
+ "pos_z": 87.40625,
+ "stations": "Sucharitkul City,Bischoff Orbital,Wiener City,Kanwar Horizons,Shunn's Claim,Capek Gateway,Bass Terminal,Gulyaev Enterprise,Amundsen Ring,Divis Silo"
+ },
+ {
+ "name": "Ojibway",
+ "pos_x": -37.03125,
+ "pos_y": -21.46875,
+ "pos_z": 24.0625,
+ "stations": "Chargaff Orbital,Xiaoguan Settlement,Walker Refinery,Bykovsky Settlement"
+ },
+ {
+ "name": "Okinoukhe",
+ "pos_x": -111.5625,
+ "pos_y": 8.84375,
+ "pos_z": -41.65625,
+ "stations": "Mendeleev Enterprise,Oxley Landing,Kovalevsky Ring,Macdonald Silo,Fincke Park"
+ },
+ {
+ "name": "Okinura",
+ "pos_x": 11.875,
+ "pos_y": 0.375,
+ "pos_z": -30.25,
+ "stations": "Bennett Gateway,Stafford Enterprise,Thorne Station,Fisher Terminal,Wright Station,Leeuwenhoek Port,Worden City,Akiyama Gateway,Dunbar Survey,Smith Forum,Volk Works,Korniyenko Terminal,Okorafor Market"
+ },
+ {
+ "name": "Okona",
+ "pos_x": -120.78125,
+ "pos_y": 61.03125,
+ "pos_z": 101.65625,
+ "stations": "Hyecho Freeport"
+ },
+ {
+ "name": "Okond",
+ "pos_x": -106.78125,
+ "pos_y": -14.28125,
+ "pos_z": -120.8125,
+ "stations": "Kuttner Settlement,Webb Horizons"
+ },
+ {
+ "name": "Okonoharyi",
+ "pos_x": 142,
+ "pos_y": -79.8125,
+ "pos_z": 40.84375,
+ "stations": "Bolton Dock,Axon Survey"
+ },
+ {
+ "name": "Okonos",
+ "pos_x": -79.03125,
+ "pos_y": 27.53125,
+ "pos_z": 113.1875,
+ "stations": "Bacon Port,Gaultier de Varennes Beacon,Archimedes Orbital"
+ },
+ {
+ "name": "Okua'gsa",
+ "pos_x": 97.375,
+ "pos_y": -127.5625,
+ "pos_z": 80.78125,
+ "stations": "Zwicky Terminal,Poyser's Folly,Matthews Terminal"
+ },
+ {
+ "name": "Okuapang",
+ "pos_x": -84.3125,
+ "pos_y": 52.8125,
+ "pos_z": -14.875,
+ "stations": "Levchenko Plant,O'Connor City,Mukai Silo,Shapiro Point"
+ },
+ {
+ "name": "Okundu",
+ "pos_x": 110.84375,
+ "pos_y": -118.90625,
+ "pos_z": 131.40625,
+ "stations": "Flammarion Port,Zhukovsky Hub,Hertzsprung Hub,Hutchinson Refinery"
+ },
+ {
+ "name": "Olcadri",
+ "pos_x": -141.8125,
+ "pos_y": 71.15625,
+ "pos_z": 20.25,
+ "stations": "Crown Station,Boyle City,Frick Landing,Lee's Folly,LeConte's Inheritance"
+ },
+ {
+ "name": "Olcae",
+ "pos_x": 107.59375,
+ "pos_y": -73.5625,
+ "pos_z": -20.34375,
+ "stations": "Severin Dock,Giacobini Vision,Turtledove Landing,Jones Gateway,Cummings Holdings"
+ },
+ {
+ "name": "Olela",
+ "pos_x": -7.5625,
+ "pos_y": 149.21875,
+ "pos_z": -33.46875,
+ "stations": "Wisniewski-Snerg Landing,al-Haytham Oasis"
+ },
+ {
+ "name": "Olelatha",
+ "pos_x": 202.34375,
+ "pos_y": -155.1875,
+ "pos_z": 41.5625,
+ "stations": "Foster Survey"
+ },
+ {
+ "name": "Olelbanu",
+ "pos_x": -83.4375,
+ "pos_y": 80.0625,
+ "pos_z": 36.5,
+ "stations": "Beckman Works"
+ },
+ {
+ "name": "Olelbis",
+ "pos_x": 89.15625,
+ "pos_y": 3.46875,
+ "pos_z": 45.1875,
+ "stations": "Polyakov Orbital,Bradbury Point,Tayler Survey,Stebler Hub,Savery Gateway"
+ },
+ {
+ "name": "Oleles",
+ "pos_x": -66.5,
+ "pos_y": -88.59375,
+ "pos_z": -103.65625,
+ "stations": "Spring Orbital,Levi-Strauss Gateway,McHugh Base"
+ },
+ {
+ "name": "Oleleuts",
+ "pos_x": -64.875,
+ "pos_y": 1.71875,
+ "pos_z": 100.375,
+ "stations": "Gidzenko Enterprise,Issigonis Port,Bean Station,Magnus Penal colony"
+ },
+ {
+ "name": "Olgrea",
+ "pos_x": -28.125,
+ "pos_y": 68.28125,
+ "pos_z": -6.375,
+ "stations": "Nearchus Terminal,Warner Station,H. G. Wells Terminal,Kratman Terminal,Asaro Installation,Berezin Port,Spinrad Enterprise,Crumey Station,Bertin Works,McCulley Installation"
+ },
+ {
+ "name": "Olker",
+ "pos_x": 46.90625,
+ "pos_y": 81.1875,
+ "pos_z": -8.46875,
+ "stations": "Hovgaard City,Dorsey Port,Nobel Base"
+ },
+ {
+ "name": "Olkolo",
+ "pos_x": 71.84375,
+ "pos_y": -48.875,
+ "pos_z": -76.28125,
+ "stations": "Sheffield Mines,Capek's Claim,Morris Survey"
+ },
+ {
+ "name": "Olkondi",
+ "pos_x": 145.21875,
+ "pos_y": -26.59375,
+ "pos_z": 30.46875,
+ "stations": "Herbert Vision,Harper Landing,Vinge Dock"
+ },
+ {
+ "name": "Ollae",
+ "pos_x": 64.84375,
+ "pos_y": -160.65625,
+ "pos_z": -45.59375,
+ "stations": "Heiles Keep,Smyth Terminal"
+ },
+ {
+ "name": "Ollavs",
+ "pos_x": -138.84375,
+ "pos_y": 38.3125,
+ "pos_z": 41.0625,
+ "stations": "Kotzebue Legacy,Cabot Landing,Gwynn's Folly"
+ },
+ {
+ "name": "Oloduma",
+ "pos_x": 133.65625,
+ "pos_y": 40.59375,
+ "pos_z": 41.75,
+ "stations": "Lazarev Enterprise,Blodgett Works"
+ },
+ {
+ "name": "Olodumanpa",
+ "pos_x": -104.09375,
+ "pos_y": -23.28125,
+ "pos_z": -116.0625,
+ "stations": "Kronecker Prospect,Nearchus Port,Gregory Holdings"
+ },
+ {
+ "name": "Olodyang",
+ "pos_x": -63.3125,
+ "pos_y": -43.5,
+ "pos_z": 41.40625,
+ "stations": "Mills Settlement"
+ },
+ {
+ "name": "Oloku",
+ "pos_x": 164.0625,
+ "pos_y": -115.75,
+ "pos_z": 18.34375,
+ "stations": "Palisa Orbital,Maitz Survey,Bond Station,Foss Depot"
+ },
+ {
+ "name": "Olokulu",
+ "pos_x": 27.75,
+ "pos_y": -108.875,
+ "pos_z": -89.28125,
+ "stations": "Schroeder Terminal,Matheson Terminal"
+ },
+ {
+ "name": "Ololones",
+ "pos_x": 134.25,
+ "pos_y": 23.75,
+ "pos_z": -61.21875,
+ "stations": "Battuta Hangar,Burnell Base"
+ },
+ {
+ "name": "Ololoney",
+ "pos_x": -64.46875,
+ "pos_y": -103.65625,
+ "pos_z": 104.03125,
+ "stations": "Babcock Dock,Roche Station"
+ },
+ {
+ "name": "Ololos",
+ "pos_x": 105.65625,
+ "pos_y": 47.0625,
+ "pos_z": 22.96875,
+ "stations": "Ramon Relay"
+ },
+ {
+ "name": "Olorun",
+ "pos_x": 37.90625,
+ "pos_y": 40.625,
+ "pos_z": -27.25,
+ "stations": "Hedley Platform"
+ },
+ {
+ "name": "Oluf",
+ "pos_x": 26.65625,
+ "pos_y": -72.9375,
+ "pos_z": 50.1875,
+ "stations": "Cabot Hub,Ayerdhal Port,Nehsi Dock,Lambert Dock"
+ },
+ {
+ "name": "Oluri",
+ "pos_x": 66.53125,
+ "pos_y": -34.25,
+ "pos_z": -143.375,
+ "stations": "Fidalgo Orbital,Marlowe Vista"
+ },
+ {
+ "name": "Oluroana",
+ "pos_x": 99.90625,
+ "pos_y": 16.3125,
+ "pos_z": -18.625,
+ "stations": "Ray Bastion,Moisuc Colony,Worlidge Point"
+ },
+ {
+ "name": "Olurorai",
+ "pos_x": -111.84375,
+ "pos_y": 114.25,
+ "pos_z": -13.96875,
+ "stations": "Bordage Arena"
+ },
+ {
+ "name": "Olurung",
+ "pos_x": 32.9375,
+ "pos_y": -27.21875,
+ "pos_z": -71.40625,
+ "stations": "McNair Mines"
+ },
+ {
+ "name": "Olwain",
+ "pos_x": -35.53125,
+ "pos_y": 73.78125,
+ "pos_z": -40.34375,
+ "stations": "Cabot Hub,Lanier's Folly,Hovell Orbital,Stjepan Seljan Terminal"
+ },
+ {
+ "name": "Omagatae",
+ "pos_x": -119.53125,
+ "pos_y": -6.9375,
+ "pos_z": -33.15625,
+ "stations": "Hiroyuki Vision,Quinn Port,Hovell Vision,Spassky Vision,Hoften Prospect,Witt Settlement"
+ },
+ {
+ "name": "Omagua",
+ "pos_x": 100.15625,
+ "pos_y": 32,
+ "pos_z": 100.3125,
+ "stations": "Panshin Dock,Andrews Dock,Ferguson Station"
+ },
+ {
+ "name": "Oman",
+ "pos_x": -140.15625,
+ "pos_y": 37.125,
+ "pos_z": 10.6875,
+ "stations": "Kuhn Horizons,Doi Orbital,Diesel Prospect,Springer Base,Lopez de Legazpi Observatory"
+ },
+ {
+ "name": "Omana",
+ "pos_x": 210.34375,
+ "pos_y": -116.96875,
+ "pos_z": 88.4375,
+ "stations": "Condit's Claim,Dugan Oasis"
+ },
+ {
+ "name": "Omanari",
+ "pos_x": 27.40625,
+ "pos_y": -17.375,
+ "pos_z": -123.53125,
+ "stations": "Roberts City"
+ },
+ {
+ "name": "Omani",
+ "pos_x": 3.84375,
+ "pos_y": 58.65625,
+ "pos_z": -59.71875,
+ "stations": "Lovell Vision,Lanchester Enterprise,Stableford Installation,Kuttner Installation,Creighton Installation"
+ },
+ {
+ "name": "Omanmatho",
+ "pos_x": -157.53125,
+ "pos_y": 20.96875,
+ "pos_z": -29.46875,
+ "stations": "Vries Gateway,O'Donnell Beacon"
+ },
+ {
+ "name": "Ombiko",
+ "pos_x": 81.71875,
+ "pos_y": -87.9375,
+ "pos_z": 6.59375,
+ "stations": "Paola Plant,Clebsch Survey"
+ },
+ {
+ "name": "Ombilia",
+ "pos_x": 15.3125,
+ "pos_y": -15.59375,
+ "pos_z": 94.75,
+ "stations": "Faraday Outpost,Roentgen Depot,Pohl Settlement"
+ },
+ {
+ "name": "Omega Sector VE-Q b5-15",
+ "pos_x": -1444.3125,
+ "pos_y": -85.8125,
+ "pos_z": 5319.9375,
+ "stations": "Omega Mining Operation"
+ },
+ {
+ "name": "Omega-1 Aquarii",
+ "pos_x": -47.125,
+ "pos_y": -132.90625,
+ "pos_z": 18.28125,
+ "stations": "Marusek Horizons,McArthur Port,Yano Point,Pratchett Observatory"
+ },
+ {
+ "name": "Omicron Capricorni B",
+ "pos_x": -52.65625,
+ "pos_y": -69,
+ "pos_z": 105.40625,
+ "stations": "Martin Enterprise,Brunel City,Litke Installation"
+ },
+ {
+ "name": "Omicron Columbae",
+ "pos_x": 75.59375,
+ "pos_y": -57.84375,
+ "pos_z": -46.28125,
+ "stations": "Baker Point,Alexander Dock,Sargent Observatory"
+ },
+ {
+ "name": "Omicron Gruis",
+ "pos_x": 25.84375,
+ "pos_y": -87.28125,
+ "pos_z": 42.28125,
+ "stations": "Niijima Gateway,Verrier Terminal,Francisco de Eliza Prospect,Shajn Port,Korolev Hub,Ziegel Orbital,Belyavsky Terminal,Keldysh Legacy,Sopwith Port,Emshwiller City,Gonnessiat Settlement,Glass Port"
+ },
+ {
+ "name": "Omicron Ursae Majoris",
+ "pos_x": -58.75,
+ "pos_y": 105.125,
+ "pos_z": -132.59375,
+ "stations": "Asimov Dock,Levy Station,So-yeon Landing"
+ },
+ {
+ "name": "Omicron-2 Eridani",
+ "pos_x": 4.59375,
+ "pos_y": -9.90625,
+ "pos_z": -12.0625,
+ "stations": "Hodgkinson Station,Polansky Barracks,Reed City"
+ },
+ {
+ "name": "Omukate",
+ "pos_x": 135.3125,
+ "pos_y": -5.96875,
+ "pos_z": 54.09375,
+ "stations": "Berezovoy Terminal"
+ },
+ {
+ "name": "Omukula",
+ "pos_x": -19.6875,
+ "pos_y": -157.90625,
+ "pos_z": 14.9375,
+ "stations": "Wirtanen Orbital,Hyakutake Enterprise,Semeonis Survey,Kopal Terminal,Plucker Survey"
+ },
+ {
+ "name": "Omumba",
+ "pos_x": 170.59375,
+ "pos_y": -91.65625,
+ "pos_z": -43.5625,
+ "stations": "Gamow Orbital,Frechet Penal colony,Zamyatin Horizons,Herbig Port"
+ },
+ {
+ "name": "Omumbojas",
+ "pos_x": -79.375,
+ "pos_y": 12.15625,
+ "pos_z": 24.53125,
+ "stations": "Thirsk Relay,Song Settlement"
+ },
+ {
+ "name": "Onateru",
+ "pos_x": -153.625,
+ "pos_y": -15,
+ "pos_z": 38.6875,
+ "stations": "Narvaez Orbital,Laird Reach"
+ },
+ {
+ "name": "Onati",
+ "pos_x": -34.84375,
+ "pos_y": -96.4375,
+ "pos_z": 68.875,
+ "stations": "Dyson Platform,Macarthur Station"
+ },
+ {
+ "name": "Ondi",
+ "pos_x": 83.3125,
+ "pos_y": -71.3125,
+ "pos_z": 85,
+ "stations": "Jeans City,Lindblad Dock,Birkhoff Installation,Molyneux Hub"
+ },
+ {
+ "name": "Ondumbo",
+ "pos_x": -86.5,
+ "pos_y": 117.3125,
+ "pos_z": -43.375,
+ "stations": "Bridges Outpost,Porsche Beacon,Vonarburg Enterprise"
+ },
+ {
+ "name": "Onduwatya",
+ "pos_x": -119.9375,
+ "pos_y": 60.34375,
+ "pos_z": -38.71875,
+ "stations": "Chilton Vision,Kier Enterprise,Hoshi Dock,Cooper Keep,Andrews Plant"
+ },
+ {
+ "name": "Ongerinja",
+ "pos_x": -40.4375,
+ "pos_y": 14.1875,
+ "pos_z": 35.84375,
+ "stations": "Vogel Platform"
+ },
+ {
+ "name": "Ongkadja",
+ "pos_x": 28.9375,
+ "pos_y": -77.375,
+ "pos_z": 106.8125,
+ "stations": "Nakano Mine,Fraknoi Barracks"
+ },
+ {
+ "name": "Ongkala",
+ "pos_x": -84.8125,
+ "pos_y": 91,
+ "pos_z": -10,
+ "stations": "Lukyanenko Orbital,Gann Base,Rochon Horizons,Humphreys Relay"
+ },
+ {
+ "name": "Ongkama",
+ "pos_x": 56.5,
+ "pos_y": -160.46875,
+ "pos_z": -87.28125,
+ "stations": "Very Colony,Alphonsi Keep,Otomo Platform,Hume Lab"
+ },
+ {
+ "name": "Ongkampan",
+ "pos_x": -49.21875,
+ "pos_y": 16.9375,
+ "pos_z": 67.59375,
+ "stations": "Carstensz Dock,Abernathy Gateway,Fawcett Gateway,Rawat Hub,Patterson Station,Ehrenfried Kegel Port,Ross Station,Rayhan al-Biruni Landing,Vercors Orbital,Watts Holdings,Goonan Prospect,Dobrovolski Beacon,Thompson Station"
+ },
+ {
+ "name": "Ongkandjeri",
+ "pos_x": 53.5625,
+ "pos_y": -71.21875,
+ "pos_z": -80.71875,
+ "stations": "Eckford Camp,Hobaugh Mines"
+ },
+ {
+ "name": "Ongkara",
+ "pos_x": 21.34375,
+ "pos_y": -56.5,
+ "pos_z": 111.28125,
+ "stations": "Naubakht Settlement,Kuiper Installation"
+ },
+ {
+ "name": "Ongkarango",
+ "pos_x": -137.90625,
+ "pos_y": -58.8125,
+ "pos_z": -25,
+ "stations": "Leonov City,Kronecker Holdings"
+ },
+ {
+ "name": "Ongkatavi",
+ "pos_x": -14.78125,
+ "pos_y": -67.625,
+ "pos_z": -110.875,
+ "stations": "Nye Mines"
+ },
+ {
+ "name": "Ongkuma",
+ "pos_x": 71.40625,
+ "pos_y": -73.4375,
+ "pos_z": 35.90625,
+ "stations": "Pickering Ring,Skiff Penal colony,Szameit Laboratory,Kirshner Relay"
+ },
+ {
+ "name": "Onil",
+ "pos_x": 101.46875,
+ "pos_y": -150.40625,
+ "pos_z": 151.78125,
+ "stations": "Scotti Vision,Takahashi Park,Goryu Station,Zillig Survey"
+ },
+ {
+ "name": "Onileut",
+ "pos_x": 124.9375,
+ "pos_y": -13.375,
+ "pos_z": 17.625,
+ "stations": "Metcalf Landing,Gresley Installation"
+ },
+ {
+ "name": "Onuras",
+ "pos_x": 125.125,
+ "pos_y": -77.96875,
+ "pos_z": 87.96875,
+ "stations": "Kuiper Station"
+ },
+ {
+ "name": "Onuris",
+ "pos_x": -9.875,
+ "pos_y": 9.6875,
+ "pos_z": 154.4375,
+ "stations": "Stokes Dock,Nielsen Horizons"
+ },
+ {
+ "name": "Onuro",
+ "pos_x": 111.4375,
+ "pos_y": -214.46875,
+ "pos_z": 88.5625,
+ "stations": "Kushida Dock"
+ },
+ {
+ "name": "Onurongo",
+ "pos_x": -103.90625,
+ "pos_y": 32.875,
+ "pos_z": 79.8125,
+ "stations": "Pudwill Gorie City,Slusser Landing"
+ },
+ {
+ "name": "Onuros",
+ "pos_x": 151.21875,
+ "pos_y": -35.03125,
+ "pos_z": 39.625,
+ "stations": "Penzias Dock,Smirnova Vision,Lee Installation,Moore Vision"
+ },
+ {
+ "name": "Onya",
+ "pos_x": 36.8125,
+ "pos_y": 117.875,
+ "pos_z": 39.9375,
+ "stations": "Kandel Prospect,du Fresne's Progress"
+ },
+ {
+ "name": "Onyaks",
+ "pos_x": 11.21875,
+ "pos_y": -117.15625,
+ "pos_z": 108.09375,
+ "stations": "Davies Landing,Adelman Oasis,Samos Installation,Johnson's Progress"
+ },
+ {
+ "name": "Onyaksas",
+ "pos_x": -3.28125,
+ "pos_y": -14.71875,
+ "pos_z": -95.5,
+ "stations": "Sadi Carnot Orbital,Cartwright Settlement,Vittori Settlement"
+ },
+ {
+ "name": "Onyali",
+ "pos_x": 103.09375,
+ "pos_y": -34.84375,
+ "pos_z": -67.1875,
+ "stations": "Wittgenstein Survey,Babcock Relay"
+ },
+ {
+ "name": "Onyama",
+ "pos_x": 140.5,
+ "pos_y": 18.46875,
+ "pos_z": 4.78125,
+ "stations": "Atwood Landing"
+ },
+ {
+ "name": "Onyayp",
+ "pos_x": -23.25,
+ "pos_y": 72.28125,
+ "pos_z": -165.78125,
+ "stations": "Gaiman Beacon"
+ },
+ {
+ "name": "Opala",
+ "pos_x": -25.5,
+ "pos_y": 35.25,
+ "pos_z": 9.28125,
+ "stations": "Onizuka's Hold,Zamka Platform,Romanenko Estate,Anderson Forum"
+ },
+ {
+ "name": "Opet",
+ "pos_x": 87.25,
+ "pos_y": -85.71875,
+ "pos_z": -51.5,
+ "stations": "Carroll Station,Pogson Gateway,Nomura Barracks,Herbig Terminal"
+ },
+ {
+ "name": "Opetani",
+ "pos_x": -145.4375,
+ "pos_y": 49.375,
+ "pos_z": 60.28125,
+ "stations": "Curie Hub,Mukai Terminal,Neumann Port"
+ },
+ {
+ "name": "Opete",
+ "pos_x": 185.71875,
+ "pos_y": -145.96875,
+ "pos_z": 22.34375,
+ "stations": "Tietjen Outpost,Albategnius Terminal,Greenland Relay"
+ },
+ {
+ "name": "Opeten",
+ "pos_x": 92.25,
+ "pos_y": 96.65625,
+ "pos_z": -4.71875,
+ "stations": "Sawyer Freeport"
+ },
+ {
+ "name": "Opetes",
+ "pos_x": -5.65625,
+ "pos_y": -102.6875,
+ "pos_z": -110.6875,
+ "stations": "Ziljak Hub,Resnick Settlement,Haber Port,Carpini Forum,Hillary Market"
+ },
+ {
+ "name": "Opila",
+ "pos_x": 5.46875,
+ "pos_y": -16.25,
+ "pos_z": -84.125,
+ "stations": "Temple Dock,Baird Orbital,Kennedy Port,al-Khayyam Ring,Peirce Orbital,Kube-McDowell City,Hayden Gateway,Clifford Vista,Wingqvist Enterprise,Barbosa Holdings"
+ },
+ {
+ "name": "Opones",
+ "pos_x": -92.53125,
+ "pos_y": 8.40625,
+ "pos_z": -33.84375,
+ "stations": "Pullman City,Ross' Inheritance,d'Eyncourt Hub"
+ },
+ {
+ "name": "Oponna",
+ "pos_x": -119.875,
+ "pos_y": 20.53125,
+ "pos_z": 26.46875,
+ "stations": "Conway Station"
+ },
+ {
+ "name": "Oponner",
+ "pos_x": 78.15625,
+ "pos_y": -54.84375,
+ "pos_z": -69.96875,
+ "stations": "Spedding Depot,Piaget Terminal"
+ },
+ {
+ "name": "Opontani",
+ "pos_x": 35.59375,
+ "pos_y": 99.03125,
+ "pos_z": -106.5,
+ "stations": "Tenn Terminal,Morgan Port,Capek Port,VanderMeer's Folly,Panshin Base"
+ },
+ {
+ "name": "Oppi",
+ "pos_x": 3.6875,
+ "pos_y": -44.625,
+ "pos_z": 131,
+ "stations": "Reiter City,Wyeth Hub"
+ },
+ {
+ "name": "Oppida",
+ "pos_x": 37.46875,
+ "pos_y": -78.4375,
+ "pos_z": 172.71875,
+ "stations": "Wenzel Hub,Meinel Station"
+ },
+ {
+ "name": "Oppidavasti",
+ "pos_x": 151.8125,
+ "pos_y": -183.5625,
+ "pos_z": 47.96875,
+ "stations": "Arfstrom Station,Lindemann Colony,Juan de la Cierva Station"
+ },
+ {
+ "name": "Oppidii",
+ "pos_x": 123.65625,
+ "pos_y": 35.96875,
+ "pos_z": -4.03125,
+ "stations": "Spielberg Port,RenenBellot Refinery,Beaumont Base"
+ },
+ {
+ "name": "OR Delphini",
+ "pos_x": -100.1875,
+ "pos_y": -34.71875,
+ "pos_z": 53.84375,
+ "stations": "Abel Hub,Leinster Gateway,Lavrador Dock,Staden Installation,Salak Reach,Stableford Orbital,Lichtenberg Station,Kovalevskaya Enterprise,Silves City,Drummond Port,Barnes Orbital,Stevens Holdings"
+ },
+ {
+ "name": "Orang",
+ "pos_x": 82.9375,
+ "pos_y": -86.28125,
+ "pos_z": 55.375,
+ "stations": "Bessel Gateway,Gregory Silo,Argelander Vision,Dilworth City,Qureshi Dock,Safdie Terminal,Irens Works,Fuchs Vision"
+ },
+ {
+ "name": "Oranito",
+ "pos_x": -48.3125,
+ "pos_y": 42.0625,
+ "pos_z": 159.03125,
+ "stations": "Parazynski Beacon,Onufrienko Base"
+ },
+ {
+ "name": "Orannika",
+ "pos_x": 53.65625,
+ "pos_y": -72.25,
+ "pos_z": -17.65625,
+ "stations": "Kagawa Dock,Hildebrandt City,Suntzeff Port,Faber Vision,Melotte Gateway,Lie Silo,Lundmark Terminal,Banach Vision,Garrido Arena"
+ },
+ {
+ "name": "Oranyayp",
+ "pos_x": 18.34375,
+ "pos_y": -191.34375,
+ "pos_z": 43.15625,
+ "stations": "Kirshner Dock"
+ },
+ {
+ "name": "Orao",
+ "pos_x": 136.03125,
+ "pos_y": -208.53125,
+ "pos_z": -49.9375,
+ "stations": "Pollas Beacon,Bisson's Progress,Phillips Platform"
+ },
+ {
+ "name": "Oraon",
+ "pos_x": 56.5,
+ "pos_y": 96.84375,
+ "pos_z": -47.8125,
+ "stations": "Quaglia Dock,Popov City"
+ },
+ {
+ "name": "Oraona",
+ "pos_x": 184.9375,
+ "pos_y": -122.71875,
+ "pos_z": 46.4375,
+ "stations": "Brera Works,Hague Depot"
+ },
+ {
+ "name": "Orcus",
+ "pos_x": 11.125,
+ "pos_y": -84.625,
+ "pos_z": 79.65625,
+ "stations": "Tombaugh Station,Oort Gateway,Schmidt Terminal,Ohain Beacon,Hovgaard Plant"
+ },
+ {
+ "name": "Ordonner",
+ "pos_x": 130.03125,
+ "pos_y": -85.65625,
+ "pos_z": 92.0625,
+ "stations": "Amano Beacon"
+ },
+ {
+ "name": "Ordovii",
+ "pos_x": 129.40625,
+ "pos_y": 108.84375,
+ "pos_z": 69.40625,
+ "stations": "Lynn Gateway,Martinez Terminal,Platt Dock,Tilman Hub,Leiber Depot"
+ },
+ {
+ "name": "Orejon",
+ "pos_x": 52,
+ "pos_y": -56.96875,
+ "pos_z": 139.0625,
+ "stations": "Leclerc Landing"
+ },
+ {
+ "name": "Orerve",
+ "pos_x": 74.03125,
+ "pos_y": 48.75,
+ "pos_z": 64.75,
+ "stations": "Watson Station"
+ },
+ {
+ "name": "Oresqu",
+ "pos_x": 57.875,
+ "pos_y": 45.84375,
+ "pos_z": 46.90625,
+ "stations": "Crumey Depot,Libby Horizons,Hoard Holdings,Deb Colony,Powers Landing"
+ },
+ {
+ "name": "Oret",
+ "pos_x": -50.09375,
+ "pos_y": 150.46875,
+ "pos_z": 78.78125,
+ "stations": "Meaney Dock"
+ },
+ {
+ "name": "Oretana",
+ "pos_x": 32.40625,
+ "pos_y": 177.46875,
+ "pos_z": 49.125,
+ "stations": "Lindbohm Retreat,MacLean Settlement"
+ },
+ {
+ "name": "Oretes",
+ "pos_x": -125.65625,
+ "pos_y": -14.09375,
+ "pos_z": -91.21875,
+ "stations": "Judson Enterprise,Amis Silo"
+ },
+ {
+ "name": "Orgelal",
+ "pos_x": 45.34375,
+ "pos_y": -52.15625,
+ "pos_z": -48.90625,
+ "stations": "Sherrington Landing,Vishweswarayya Penitentiary,Whitney Terminal"
+ },
+ {
+ "name": "Orgeleu",
+ "pos_x": 120.15625,
+ "pos_y": -123.15625,
+ "pos_z": -20.75,
+ "stations": "Gurevich Landing,Kawanishi Installation"
+ },
+ {
+ "name": "Orgelma",
+ "pos_x": 110,
+ "pos_y": -10.0625,
+ "pos_z": -113.21875,
+ "stations": "Nylund Port"
+ },
+ {
+ "name": "Orgen",
+ "pos_x": 165.46875,
+ "pos_y": -4.84375,
+ "pos_z": 79.3125,
+ "stations": "Pribylov City,Dukaj Hub,Block Installation,Fancher Station"
+ },
+ {
+ "name": "Orgetu",
+ "pos_x": -92.96875,
+ "pos_y": -25.1875,
+ "pos_z": -75.96875,
+ "stations": "Lenoir Colony"
+ },
+ {
+ "name": "Ori",
+ "pos_x": -26.28125,
+ "pos_y": 56.09375,
+ "pos_z": 2.4375,
+ "stations": "Taylor Survey,McIntyre's Progress,Dedekind Terminal"
+ },
+ {
+ "name": "Oris",
+ "pos_x": -82.78125,
+ "pos_y": -97.84375,
+ "pos_z": -21.21875,
+ "stations": "Ramsay Hub"
+ },
+ {
+ "name": "Orisala",
+ "pos_x": -84.59375,
+ "pos_y": 1.8125,
+ "pos_z": -88,
+ "stations": "Kidman Landing"
+ },
+ {
+ "name": "Orisater",
+ "pos_x": 69.09375,
+ "pos_y": -11.0625,
+ "pos_z": 135.8125,
+ "stations": "Larson Orbital,Hunt Terminal"
+ },
+ {
+ "name": "Orisattu",
+ "pos_x": 104.53125,
+ "pos_y": -4.4375,
+ "pos_z": 122.3125,
+ "stations": "Wallin Point,Godwin Terminal,Rice Beacon"
+ },
+ {
+ "name": "Orishala",
+ "pos_x": -30.09375,
+ "pos_y": -79.34375,
+ "pos_z": -55.5,
+ "stations": "Yu Hub,Santarem Orbital,Bentham Platform"
+ },
+ {
+ "name": "Orishalina",
+ "pos_x": -136.75,
+ "pos_y": 35.25,
+ "pos_z": 92.875,
+ "stations": "Block Orbital,Thompson Installation,Janes Settlement,Galois Settlement"
+ },
+ {
+ "name": "Orishanann",
+ "pos_x": -88.28125,
+ "pos_y": -82.28125,
+ "pos_z": -63,
+ "stations": "Cortes Landing,Hawkes Beacon,Stefansson Station"
+ },
+ {
+ "name": "Orishanh",
+ "pos_x": -113,
+ "pos_y": -49.125,
+ "pos_z": 24.59375,
+ "stations": "Macdonald Platform,Marley's Claim,Robinson Terminal"
+ },
+ {
+ "name": "Orishiali",
+ "pos_x": -63.8125,
+ "pos_y": -99.6875,
+ "pos_z": 100.5625,
+ "stations": "Hand Platform"
+ },
+ {
+ "name": "Orishis",
+ "pos_x": -31,
+ "pos_y": 93.96875,
+ "pos_z": -3.5625,
+ "stations": "Chasles Base,Piccard Dock"
+ },
+ {
+ "name": "Orishpucho",
+ "pos_x": 59.15625,
+ "pos_y": 20.6875,
+ "pos_z": -37.90625,
+ "stations": "Beregovoi Colony,Reiter Colony,Cockrell City,Young Arsenal"
+ },
+ {
+ "name": "Orishvati",
+ "pos_x": -8.03125,
+ "pos_y": 15.90625,
+ "pos_z": 77.84375,
+ "stations": "Harrison Orbital,Mille Dock,Robinson Horizons,Hiroyuki Depot"
+ },
+ {
+ "name": "Orisini",
+ "pos_x": 105.78125,
+ "pos_y": 66.78125,
+ "pos_z": -106.40625,
+ "stations": "Starzl Dock,Wait Colburn Base,Alenquer Freeport,Andersson Prospect,Barentsz Base"
+ },
+ {
+ "name": "Orniaci",
+ "pos_x": -153.75,
+ "pos_y": -43.78125,
+ "pos_z": 15.9375,
+ "stations": "Lorrah Installation,White Bastion"
+ },
+ {
+ "name": "Ornicornat",
+ "pos_x": -4.75,
+ "pos_y": -120.3125,
+ "pos_z": -81.90625,
+ "stations": "Burnell Platform,Blaha Colony,Kippax Landing"
+ },
+ {
+ "name": "Orobriambu",
+ "pos_x": -71.78125,
+ "pos_y": 67.34375,
+ "pos_z": -137.625,
+ "stations": "Chadwick Port,Yang Keep,Kaleri Platform"
+ },
+ {
+ "name": "Orobriges",
+ "pos_x": 18.34375,
+ "pos_y": 95.59375,
+ "pos_z": -102.28125,
+ "stations": "Turzillo's Claim,Redi Platform"
+ },
+ {
+ "name": "Orobrogee",
+ "pos_x": 78.3125,
+ "pos_y": 61.5,
+ "pos_z": -123.46875,
+ "stations": "Fairbairn Platform,Haldeman Platform,Mitchell Laboratory"
+ },
+ {
+ "name": "Orobu",
+ "pos_x": -144.4375,
+ "pos_y": -20.28125,
+ "pos_z": 67.90625,
+ "stations": "Malyshev Station,Birkeland Station,Winne Orbital,Overmyer Barracks"
+ },
+ {
+ "name": "Orocori",
+ "pos_x": -6.28125,
+ "pos_y": -120.125,
+ "pos_z": 81.65625,
+ "stations": "Fabian Installation,Saarinen Landing,Brundage Estate"
+ },
+ {
+ "name": "Orokudumbla",
+ "pos_x": -6.5,
+ "pos_y": -16.8125,
+ "pos_z": 195.53125,
+ "stations": "Palmer Prospect,Navigator Landing"
+ },
+ {
+ "name": "Orom",
+ "pos_x": 95.5625,
+ "pos_y": -19.78125,
+ "pos_z": -54.59375,
+ "stations": "Sacco Landing"
+ },
+ {
+ "name": "Orom Djugua",
+ "pos_x": 74.34375,
+ "pos_y": -67.03125,
+ "pos_z": 70.78125,
+ "stations": "Niemeyer Beacon,Roed Odegaard Terminal"
+ },
+ {
+ "name": "Oromecani",
+ "pos_x": 129.25,
+ "pos_y": 16.0625,
+ "pos_z": 93.5625,
+ "stations": "Stanley Platform,Rond d'Alembert Colony,Margulies Oasis"
+ },
+ {
+ "name": "Oromila",
+ "pos_x": -152.5,
+ "pos_y": -70.9375,
+ "pos_z": 16.40625,
+ "stations": "Stasheff Dock,McDevitt Reformatory"
+ },
+ {
+ "name": "Orong",
+ "pos_x": -103,
+ "pos_y": 68.34375,
+ "pos_z": -72.375,
+ "stations": "Savinykh Landing,Volta Stop"
+ },
+ {
+ "name": "Oronitek",
+ "pos_x": 105.40625,
+ "pos_y": 113.4375,
+ "pos_z": -55.71875,
+ "stations": "Levchenko Refinery,Landsteiner Settlement"
+ },
+ {
+ "name": "Orrere",
+ "pos_x": 68.84375,
+ "pos_y": 48.75,
+ "pos_z": 76.75,
+ "stations": "Walker's Progress,Guin Survey,Sharon Lee Free Market,Forward Horizons,Kimbrough's Folly,Ahmed Enterprise"
+ },
+ {
+ "name": "Orulanqui",
+ "pos_x": 60.3125,
+ "pos_y": 100.25,
+ "pos_z": -25.4375,
+ "stations": "Boulle Port,Haber Gateway,Sladek Orbital,Stein Port,Bernoulli Hub,Harper Port,Heaviside Station"
+ },
+ {
+ "name": "Orulas",
+ "pos_x": 76.75,
+ "pos_y": -141.375,
+ "pos_z": -120.375,
+ "stations": "Converse Platform"
+ },
+ {
+ "name": "Orule",
+ "pos_x": 84.0625,
+ "pos_y": -84.8125,
+ "pos_z": 94.4375,
+ "stations": "Huss Refinery,Baker Settlement,Miyasaka Holdings"
+ },
+ {
+ "name": "Orulebo",
+ "pos_x": 67.3125,
+ "pos_y": -70.5625,
+ "pos_z": 79.15625,
+ "stations": "Hevelius Depot"
+ },
+ {
+ "name": "Orulendiji",
+ "pos_x": 137.25,
+ "pos_y": -142.21875,
+ "pos_z": 42.53125,
+ "stations": "Meinel Installation,Husband Camp,Russell Settlement,Zhu Settlement"
+ },
+ {
+ "name": "Osage",
+ "pos_x": -16.21875,
+ "pos_y": -195.8125,
+ "pos_z": -4.375,
+ "stations": "Giclas Prospect,al-Din al-Urdi Survey,von Bellingshausen Beacon,Cowell Depot"
+ },
+ {
+ "name": "Osanartar",
+ "pos_x": 87.96875,
+ "pos_y": -60.84375,
+ "pos_z": -80.53125,
+ "stations": "Ayton Innes Port,Shirley Depot,Kier Relay,DiFate Terminal,Kuchemann Installation,Kurland Survey"
+ },
+ {
+ "name": "Osane",
+ "pos_x": 142.90625,
+ "pos_y": -154.25,
+ "pos_z": 90.65625,
+ "stations": "Bell Vision,Digges Park,Wetherill Platform"
+ },
+ {
+ "name": "Osans",
+ "pos_x": -39.40625,
+ "pos_y": 60.03125,
+ "pos_z": 76.875,
+ "stations": "Fibonacci Refinery,Kuttner Installation,Bischoff Prospect"
+ },
+ {
+ "name": "Oscabi",
+ "pos_x": 125.1875,
+ "pos_y": -16,
+ "pos_z": 18.4375,
+ "stations": "von Helmont Port,de Andrade Penal colony,Ford Holdings"
+ },
+ {
+ "name": "Oscabs",
+ "pos_x": -76.625,
+ "pos_y": -72.28125,
+ "pos_z": 84,
+ "stations": "Eyharts Port,Noriega Orbital,Linteris Terminal,Lindsey Hub,Kuo Base,Korniyenko Beacon"
+ },
+ {
+ "name": "Oscans",
+ "pos_x": 116.4375,
+ "pos_y": -73.15625,
+ "pos_z": -55.90625,
+ "stations": "Kreutz Beacon"
+ },
+ {
+ "name": "Osci",
+ "pos_x": 102.71875,
+ "pos_y": -152.53125,
+ "pos_z": -56.8125,
+ "stations": "Hague Port,Serling's Progress,Tsunenaga Base"
+ },
+ {
+ "name": "Oserktomen",
+ "pos_x": 46.59375,
+ "pos_y": 40.21875,
+ "pos_z": -113.625,
+ "stations": "Tanner Beacon"
+ },
+ {
+ "name": "Osermians",
+ "pos_x": 86.3125,
+ "pos_y": -26.09375,
+ "pos_z": -69.5,
+ "stations": "Crick Orbital,Usachov Hub,Whitney Station,Carr Hub,Irens Survey,Shepherd Base,Diesel Vista"
+ },
+ {
+ "name": "Osermo",
+ "pos_x": -4.90625,
+ "pos_y": 161,
+ "pos_z": -25.84375,
+ "stations": "Ayerdhal Survey"
+ },
+ {
+ "name": "Osha",
+ "pos_x": 127.28125,
+ "pos_y": -63.59375,
+ "pos_z": -105.75,
+ "stations": "Abasheli's Inheritance,Desargues Survey,Tavernier Works"
+ },
+ {
+ "name": "Oshambia",
+ "pos_x": 187.5625,
+ "pos_y": -131.65625,
+ "pos_z": 34.3125,
+ "stations": "Shoujing Settlement,Johnson Relay"
+ },
+ {
+ "name": "Oshanla",
+ "pos_x": 128.96875,
+ "pos_y": -148.875,
+ "pos_z": 41.09375,
+ "stations": "Reid Gateway,Parker Orbital,Folland Ring,Hickman Arsenal,Baydukov Settlement"
+ },
+ {
+ "name": "Oshatha",
+ "pos_x": 128.53125,
+ "pos_y": -220.3125,
+ "pos_z": 86.625,
+ "stations": "Hawker Oasis,Ashman Holdings,Kornbluth Reach"
+ },
+ {
+ "name": "Oshepra",
+ "pos_x": 5.21875,
+ "pos_y": 43.375,
+ "pos_z": 73.78125,
+ "stations": "Kozeyev Ring,Celsius City,Lambert Enterprise,Shunn's Progress"
+ },
+ {
+ "name": "Osira",
+ "pos_x": -92.65625,
+ "pos_y": 100.46875,
+ "pos_z": 86.0625,
+ "stations": "Thompson Enterprise,Cousteau Orbital,Cooper Ring,Slade Enterprise,Bartlett Gateway,Lee Orbital,Turtledove Works,Geston Station,Patterson Bastion,Jenkinson Laboratory"
+ },
+ {
+ "name": "Osire Fades",
+ "pos_x": 96.375,
+ "pos_y": -239.0625,
+ "pos_z": 77.5,
+ "stations": "Flammarion Terminal"
+ },
+ {
+ "name": "Osiris",
+ "pos_x": -40.78125,
+ "pos_y": 55.90625,
+ "pos_z": 74.46875,
+ "stations": "Harrison Port,Fearn Prospect,Besonders Mines"
+ },
+ {
+ "name": "Osis",
+ "pos_x": -159.21875,
+ "pos_y": -15.0625,
+ "pos_z": 53,
+ "stations": "Preuss Holdings,Gromov Installation,Salak Relay"
+ },
+ {
+ "name": "Osismii",
+ "pos_x": -66.09375,
+ "pos_y": -175.75,
+ "pos_z": 94.84375,
+ "stations": "Tilman Point"
+ },
+ {
+ "name": "Osismonti",
+ "pos_x": 129.15625,
+ "pos_y": 32.34375,
+ "pos_z": 134.46875,
+ "stations": "Hume Settlement"
+ },
+ {
+ "name": "Osissi",
+ "pos_x": 68.625,
+ "pos_y": 131.53125,
+ "pos_z": 45.84375,
+ "stations": "Hogan Ring,Hodgkinson Hub,Forfait Hub,MacLeod's Inheritance"
+ },
+ {
+ "name": "Osissi Hua",
+ "pos_x": 106.6875,
+ "pos_y": -103.84375,
+ "pos_z": 84.21875,
+ "stations": "Howe Holdings,Sutter Orbital,Lefschetz Beacon,Schade Vision"
+ },
+ {
+ "name": "Ossian",
+ "pos_x": 69.25,
+ "pos_y": -102.90625,
+ "pos_z": -100.09375,
+ "stations": "Kondo Orbital,Albumasar Holdings,Huggins Port,Byrd Works"
+ },
+ {
+ "name": "Ossians",
+ "pos_x": 103.21875,
+ "pos_y": -57.875,
+ "pos_z": -90.71875,
+ "stations": "Dirichlet Plant,Legendre Platform,McKay Penal colony"
+ },
+ {
+ "name": "Ossim",
+ "pos_x": -112.53125,
+ "pos_y": -23.53125,
+ "pos_z": 109.03125,
+ "stations": "Strzelecki's Claim"
+ },
+ {
+ "name": "Ossimava",
+ "pos_x": -51.90625,
+ "pos_y": 108.25,
+ "pos_z": -63.71875,
+ "stations": "Maxwell Holdings,Euler Hangar"
+ },
+ {
+ "name": "Ossito",
+ "pos_x": 138.09375,
+ "pos_y": -67.90625,
+ "pos_z": -6.125,
+ "stations": "Stuart Mines,Dietz Vision,Vizcaino Dock"
+ },
+ {
+ "name": "Ostya",
+ "pos_x": 48.75,
+ "pos_y": 37.5625,
+ "pos_z": -104.65625,
+ "stations": "Mitchell Settlement,Sheckley Enterprise,Garriott Colony"
+ },
+ {
+ "name": "Ostyas",
+ "pos_x": -111.15625,
+ "pos_y": 75.53125,
+ "pos_z": -45.59375,
+ "stations": "Sargent Installation,Issigonis Platform,Northrop Enterprise"
+ },
+ {
+ "name": "Ostyat",
+ "pos_x": 143.96875,
+ "pos_y": 9.65625,
+ "pos_z": 7.9375,
+ "stations": "White Terminal,Rucker Enterprise,Reed Orbital,Hyecho Orbital"
+ },
+ {
+ "name": "Osuntes",
+ "pos_x": 42.09375,
+ "pos_y": 130.21875,
+ "pos_z": -11.875,
+ "stations": "Cook Orbital,Shumil Dock,Stableford Depot,Xuanzang Hub"
+ },
+ {
+ "name": "Osuntii",
+ "pos_x": 56.6875,
+ "pos_y": -54.90625,
+ "pos_z": 194.5,
+ "stations": "Payne Terminal,Resnick Survey,Wallace Penal colony"
+ },
+ {
+ "name": "Otatii",
+ "pos_x": 167.09375,
+ "pos_y": -101.40625,
+ "pos_z": 52.34375,
+ "stations": "Lundmark's Claim"
+ },
+ {
+ "name": "Othe",
+ "pos_x": 116.875,
+ "pos_y": 10.09375,
+ "pos_z": 93.6875,
+ "stations": "Tasman Depot,Abnett Dock,Rustah Platform"
+ },
+ {
+ "name": "Othel",
+ "pos_x": 13.59375,
+ "pos_y": -93.59375,
+ "pos_z": 2.46875,
+ "stations": "Ernst Refinery,Valz Installation,Wafer Landing,Arago Holdings,Anderson Colony"
+ },
+ {
+ "name": "Othelkan",
+ "pos_x": -162.84375,
+ "pos_y": 51.59375,
+ "pos_z": -84.5,
+ "stations": "Alexandrov Landing,Dietz Relay"
+ },
+ {
+ "name": "Otherni",
+ "pos_x": 73.71875,
+ "pos_y": -62.5,
+ "pos_z": -61.125,
+ "stations": "Ryazanski Enterprise,McCulley Refinery"
+ },
+ {
+ "name": "Othet",
+ "pos_x": -72.75,
+ "pos_y": 36.96875,
+ "pos_z": 87.25,
+ "stations": "Salgari City,Hill Orbital,Hausdorff Port,Conway Refinery"
+ },
+ {
+ "name": "Othi",
+ "pos_x": 48.78125,
+ "pos_y": 56.28125,
+ "pos_z": 65.75,
+ "stations": "McMullen Prospect"
+ },
+ {
+ "name": "Othieh",
+ "pos_x": 115.71875,
+ "pos_y": 102.4375,
+ "pos_z": -29.46875,
+ "stations": "Carr Port"
+ },
+ {
+ "name": "Othime",
+ "pos_x": 2.40625,
+ "pos_y": -26.4375,
+ "pos_z": 52.4375,
+ "stations": "Levi-Montalcini Dock,Lone Rock"
+ },
+ {
+ "name": "Othit",
+ "pos_x": 120.3125,
+ "pos_y": 78.4375,
+ "pos_z": 37.5625,
+ "stations": "Constantine Enterprise,Fremont Relay,Nelder Beacon"
+ },
+ {
+ "name": "Othiti",
+ "pos_x": 47.1875,
+ "pos_y": 85.6875,
+ "pos_z": -136.4375,
+ "stations": "Kuttner Refinery,Matthews Station,Lopez de Legazpi Installation"
+ },
+ {
+ "name": "Oto",
+ "pos_x": 27.25,
+ "pos_y": -20.71875,
+ "pos_z": -47.15625,
+ "stations": "Gauss City,Wheelock Vision,Wyeth Terminal,Pauling Enterprise,Rothfuss Point,Norton Settlement"
+ },
+ {
+ "name": "Otohiko",
+ "pos_x": -57.46875,
+ "pos_y": 32.6875,
+ "pos_z": -142.21875,
+ "stations": "Glidden Terminal,Moseley Horizons,Weil Legacy,Satcher Ring,Burgess Settlement"
+ },
+ {
+ "name": "Otohime",
+ "pos_x": 191.46875,
+ "pos_y": -180.625,
+ "pos_z": 88.96875,
+ "stations": "Eggen Laboratory,Hampson Survey,Parker Orbital"
+ },
+ {
+ "name": "Otshe",
+ "pos_x": 107.21875,
+ "pos_y": 22.90625,
+ "pos_z": -20.78125,
+ "stations": "Kuo Terminal,Bosch Terminal"
+ },
+ {
+ "name": "Otshirvani",
+ "pos_x": 48.1875,
+ "pos_y": 89.3125,
+ "pos_z": -37.0625,
+ "stations": "Burke Station,Gunn Settlement,Xuanzang Station,Baird Plant,Soukup Vista"
+ },
+ {
+ "name": "Ottia",
+ "pos_x": -68.625,
+ "pos_y": 7,
+ "pos_z": -71.8125,
+ "stations": "Davy Dock,Clark Prospect,Akers Enterprise,Bresnik Terminal,Anvil Terminal"
+ },
+ {
+ "name": "OU Geminorum",
+ "pos_x": 11.34375,
+ "pos_y": 3.0625,
+ "pos_z": -46.375,
+ "stations": "Bloomfield Station,Mayr Penal colony,Gauss Terminal,Cartwright Terminal,Haignere City,Hurston Hub,Weston Orbital,Feustel Oasis,Velazquez Port,Stirling Prospect,Kozlov's Inheritance,Chargaff Enterprise,Glidden Orbital,Trinh Landing"
+ },
+ {
+ "name": "Outotz ST-I d9-6",
+ "pos_x": 604.15625,
+ "pos_y": 153.59375,
+ "pos_z": -1514.9375,
+ "stations": "The Gnosis"
+ },
+ {
+ "name": "Ovamalu",
+ "pos_x": 42.03125,
+ "pos_y": -160.8125,
+ "pos_z": -18.03125,
+ "stations": "Plancius Port,Wang Ring,Goldreich Ring,Mechain Relay"
+ },
+ {
+ "name": "Ovid",
+ "pos_x": -28.0625,
+ "pos_y": 35.15625,
+ "pos_z": 14.8125,
+ "stations": "Bradfield Orbital,Shriver Platform,Abe Works"
+ },
+ {
+ "name": "Ovimbaia",
+ "pos_x": 71.59375,
+ "pos_y": -16.21875,
+ "pos_z": 121.34375,
+ "stations": "Morris City,Preuss Plant,Phillifent Horizons,Maler Vision,Thornycroft Mines"
+ },
+ {
+ "name": "Ovimbiabas",
+ "pos_x": 23.3125,
+ "pos_y": -61.96875,
+ "pos_z": -9.0625,
+ "stations": "Nolan Hub,Kaku City,Vogel Orbital,Clarke Relay,Hopkins Enterprise"
+ },
+ {
+ "name": "Ovimbundu",
+ "pos_x": -86.4375,
+ "pos_y": 28.59375,
+ "pos_z": 61.03125,
+ "stations": "Popov Works,Kerwin Mines,Xing Relay"
+ },
+ {
+ "name": "Ovini",
+ "pos_x": 145.3125,
+ "pos_y": -133.59375,
+ "pos_z": -43.625,
+ "stations": "Swift Orbital,Abetti Station,McCool Terminal,Malaspina's Progress"
+ },
+ {
+ "name": "Ovinn",
+ "pos_x": 27.65625,
+ "pos_y": -82.5,
+ "pos_z": -89,
+ "stations": "Townshend Hub"
+ },
+ {
+ "name": "Ovinnik",
+ "pos_x": 124.75,
+ "pos_y": -76.4375,
+ "pos_z": 77.03125,
+ "stations": "Airy Landing"
+ },
+ {
+ "name": "Owionenseno",
+ "pos_x": -76.53125,
+ "pos_y": 35.40625,
+ "pos_z": 71.1875,
+ "stations": "Zalyotin Mine,Delany Camp"
+ },
+ {
+ "name": "Owiones",
+ "pos_x": 23.53125,
+ "pos_y": 108.3125,
+ "pos_z": 118.84375,
+ "stations": "Covey Works"
+ },
+ {
+ "name": "Oxlaha",
+ "pos_x": -131.0625,
+ "pos_y": -20.71875,
+ "pos_z": -54.8125,
+ "stations": "Reed Relay"
+ },
+ {
+ "name": "Oxlahatta",
+ "pos_x": -51.09375,
+ "pos_y": -126.96875,
+ "pos_z": 28.125,
+ "stations": "Fullerton Point,Ewald Orbital"
+ },
+ {
+ "name": "Oxlahuntiku",
+ "pos_x": 8.125,
+ "pos_y": 101.71875,
+ "pos_z": 30.0625,
+ "stations": "Linge Hangar,Williams Terminal,Gibson Works"
+ },
+ {
+ "name": "Oxossi",
+ "pos_x": 88.40625,
+ "pos_y": -52.5,
+ "pos_z": 27.90625,
+ "stations": "Kapteyn Hub,Shinjo Station,Eggen Prospect"
+ },
+ {
+ "name": "Oxosso Kait",
+ "pos_x": 49.1875,
+ "pos_y": -76.9375,
+ "pos_z": -100.03125,
+ "stations": "Aller Dock"
+ },
+ {
+ "name": "Oyas",
+ "pos_x": 42.53125,
+ "pos_y": 15.4375,
+ "pos_z": 50.65625,
+ "stations": "Stafford Depot,Wetherbee Orbital"
+ },
+ {
+ "name": "p Velorum",
+ "pos_x": 84.75,
+ "pos_y": 13.40625,
+ "pos_z": 17.25,
+ "stations": "Tayler Keep,Noakes Horizons"
+ },
+ {
+ "name": "P'urhep",
+ "pos_x": 177.75,
+ "pos_y": -120.21875,
+ "pos_z": 65.96875,
+ "stations": "Shinjo Camp,Busch Holdings"
+ },
+ {
+ "name": "Pa Kammai",
+ "pos_x": 95.0625,
+ "pos_y": -161.5625,
+ "pos_z": 168.34375,
+ "stations": "Hirayama Mines"
+ },
+ {
+ "name": "Pa Kazahuan",
+ "pos_x": -76.40625,
+ "pos_y": -2.625,
+ "pos_z": 106.65625,
+ "stations": "McCandless Ring,Davy Platform,Britnev Enterprise,Sutcliffe Penal colony"
+ },
+ {
+ "name": "Pa Pa Pole",
+ "pos_x": 59.25,
+ "pos_y": -0.40625,
+ "pos_z": 139.8125,
+ "stations": "Kennicott City,Jakes Port,Hahn Ring,Napier Camp,Hume's Progress"
+ },
+ {
+ "name": "Pa Para",
+ "pos_x": -76.125,
+ "pos_y": -10.75,
+ "pos_z": 78.40625,
+ "stations": "Ayerdhal Hangar,Morgan Station,Boe Holdings,Narita Keep"
+ },
+ {
+ "name": "Pa Puggsa",
+ "pos_x": -169.8125,
+ "pos_y": -16.625,
+ "pos_z": -15.90625,
+ "stations": "Silverberg Landing,Fisk Station"
+ },
+ {
+ "name": "Pacalite",
+ "pos_x": -63.4375,
+ "pos_y": -33.9375,
+ "pos_z": 34.34375,
+ "stations": "Bacigalupi Bastion,Laird Hub,Aksyonov Orbital"
+ },
+ {
+ "name": "Pacamundu",
+ "pos_x": 51.96875,
+ "pos_y": 92.5,
+ "pos_z": 85.71875,
+ "stations": "Marques Port,Menezes Station,Beliaev Landing,Banach Horizons,Garcia Enterprise"
+ },
+ {
+ "name": "Pacana",
+ "pos_x": -30.75,
+ "pos_y": 43.90625,
+ "pos_z": 42.375,
+ "stations": "Savitskaya Terminal,Bardeen Ring"
+ },
+ {
+ "name": "Pacanis",
+ "pos_x": 156.59375,
+ "pos_y": -32.25,
+ "pos_z": 5.1875,
+ "stations": "Hale Landing"
+ },
+ {
+ "name": "Pacap",
+ "pos_x": 42.0625,
+ "pos_y": 66.90625,
+ "pos_z": -40.21875,
+ "stations": "Steinmuller Orbital,Cogswell City,Morgan Port,Stefanyshyn-Piper Beacon,Gwynn's Inheritance,Chaviano Refinery"
+ },
+ {
+ "name": "Pach Kach",
+ "pos_x": 75.15625,
+ "pos_y": -159.5625,
+ "pos_z": -71.65625,
+ "stations": "Cuffey City,Shapiro Base,Cassini Bastion"
+ },
+ {
+ "name": "Pachamama",
+ "pos_x": 3.84375,
+ "pos_y": -61.8125,
+ "pos_z": 26.9375,
+ "stations": "Schottky Outpost"
+ },
+ {
+ "name": "Pachanu",
+ "pos_x": -160.21875,
+ "pos_y": 40.53125,
+ "pos_z": -55.75,
+ "stations": "Bennett Outpost,McCormick Base,Freycinet Relay"
+ },
+ {
+ "name": "Pachanwen",
+ "pos_x": 28.0625,
+ "pos_y": -13.65625,
+ "pos_z": -46.90625,
+ "stations": "Bryant Dock,Carr Station,Scott Station,Hahn Terminal,Sewell Settlement,Dukaj Bastion,MacLeod Installation"
+ },
+ {
+ "name": "Pachita",
+ "pos_x": 108.5,
+ "pos_y": 2.34375,
+ "pos_z": 44.09375,
+ "stations": "Al-Battani Ring,Bugrov Gateway"
+ },
+ {
+ "name": "Padh",
+ "pos_x": 119.75,
+ "pos_y": -241.96875,
+ "pos_z": 63.34375,
+ "stations": "Merritt Hub,Grunsfeld Orbital"
+ },
+ {
+ "name": "Padhbh",
+ "pos_x": 172.4375,
+ "pos_y": 18.65625,
+ "pos_z": 39.53125,
+ "stations": "Midgeley Landing,Hopkins Point,Brera Enterprise"
+ },
+ {
+ "name": "Padhyas",
+ "pos_x": -1.625,
+ "pos_y": -12.6875,
+ "pos_z": -85.40625,
+ "stations": "Mattingly Dock,Chapman Terminal,Reisman Port,Winne's Folly"
+ },
+ {
+ "name": "Paeligni",
+ "pos_x": -85.0625,
+ "pos_y": 1.4375,
+ "pos_z": 136.15625,
+ "stations": "Dirac Dock,Haignere Ring,Poleshchuk Landing,Hoften Terminal,Grimwood's Inheritance"
+ },
+ {
+ "name": "Paeling",
+ "pos_x": 59.125,
+ "pos_y": 11.15625,
+ "pos_z": 134.21875,
+ "stations": "Ericsson Relay"
+ },
+ {
+ "name": "Paelis",
+ "pos_x": -89.65625,
+ "pos_y": -96.59375,
+ "pos_z": -23.75,
+ "stations": "Rennie Station"
+ },
+ {
+ "name": "Paemalites",
+ "pos_x": 2.125,
+ "pos_y": -48.125,
+ "pos_z": 68.3125,
+ "stations": "Wankel Hangar,Eskridge Installation,Herrington Station"
+ },
+ {
+ "name": "Paemani",
+ "pos_x": -10.3125,
+ "pos_y": 87.03125,
+ "pos_z": -138.6875,
+ "stations": "Burkin Mine,Rasch's Folly"
+ },
+ {
+ "name": "Paemara",
+ "pos_x": 100.5,
+ "pos_y": 69.0625,
+ "pos_z": 84.25,
+ "stations": "Rukavishnikov Terminal"
+ },
+ {
+ "name": "Paeni",
+ "pos_x": 71.3125,
+ "pos_y": -58.28125,
+ "pos_z": -134.03125,
+ "stations": "Grover Orbital,Glazkov Ring,Reamy Barracks,Fujimori Enterprise"
+ },
+ {
+ "name": "Paentun",
+ "pos_x": 154.71875,
+ "pos_y": -192.125,
+ "pos_z": 70.40625,
+ "stations": "Abbot Terminal,Naburimannu Dock,Cottenot Vision,Barbosa's Progress"
+ },
+ {
+ "name": "Paesan",
+ "pos_x": -47.5,
+ "pos_y": -77.46875,
+ "pos_z": -138.59375,
+ "stations": "Abel Landing,Dietz Platform,Scott Barracks"
+ },
+ {
+ "name": "Paesans",
+ "pos_x": 134.15625,
+ "pos_y": -74.75,
+ "pos_z": 50.8125,
+ "stations": "Kuiper Gateway,Diamond Base,Chalker Relay"
+ },
+ {
+ "name": "Paesi",
+ "pos_x": -31.75,
+ "pos_y": -155.90625,
+ "pos_z": 21.40625,
+ "stations": "Nijland Port,Westerhout Holdings,Fowler Orbital,Haldeman II Silo"
+ },
+ {
+ "name": "Paesia",
+ "pos_x": 107.59375,
+ "pos_y": -15.0625,
+ "pos_z": 130.4375,
+ "stations": "Ramon City,Burgess Point,McCormick Ring,Evans Orbital"
+ },
+ {
+ "name": "Paesici",
+ "pos_x": 9.40625,
+ "pos_y": -59.21875,
+ "pos_z": 125.40625,
+ "stations": "Sellings Hub,Wilhelm Terminal,Gibbs Enterprise,Brin Enterprise,Panshin Ring,Zamyatin Station,Bunch Terminal,Freycinet Vision,Zebrowski Enterprise,Dickson Depot,Iqbal Point"
+ },
+ {
+ "name": "Paesing",
+ "pos_x": -100.28125,
+ "pos_y": -104.625,
+ "pos_z": 50.96875,
+ "stations": "Rorschach Dock,Grover Refinery,Lee Camp"
+ },
+ {
+ "name": "Paestae",
+ "pos_x": -79.96875,
+ "pos_y": -79.15625,
+ "pos_z": -140.125,
+ "stations": "Stefansson Dock,Gaiman Barracks,Crown Vision"
+ },
+ {
+ "name": "Paeste",
+ "pos_x": 127.09375,
+ "pos_y": 71.84375,
+ "pos_z": 103.4375,
+ "stations": "Vishweswarayya Dock,Merbold Station,Semeonis Vision,Hodgkin Port"
+ },
+ {
+ "name": "Paesui Xena",
+ "pos_x": 116.875,
+ "pos_y": -62.0625,
+ "pos_z": 23.375,
+ "stations": "Thollon Platform,Reeves Vision"
+ },
+ {
+ "name": "Paesvate",
+ "pos_x": 92.03125,
+ "pos_y": -169.75,
+ "pos_z": -4.8125,
+ "stations": "van der Riet Woolley Mines"
+ },
+ {
+ "name": "Pah",
+ "pos_x": 103.375,
+ "pos_y": 71.875,
+ "pos_z": -21.25,
+ "stations": "Malyshev Colony,Fisher Landing,Young's Claim"
+ },
+ {
+ "name": "Pai Ho",
+ "pos_x": 9.9375,
+ "pos_y": -94.8125,
+ "pos_z": -52.3125,
+ "stations": "Lonchakov Mines,Hardwick Survey"
+ },
+ {
+ "name": "Pai Huldr",
+ "pos_x": 52.625,
+ "pos_y": -114.59375,
+ "pos_z": 7.1875,
+ "stations": "Urkovic Station,Ryle Orbital,Luther Installation,Stuart's Progress"
+ },
+ {
+ "name": "Pai Shihil",
+ "pos_x": -134.40625,
+ "pos_y": -38.40625,
+ "pos_z": 24.78125,
+ "stations": "Fraser Dock,Haarsma Dock,Ron Hubbard Laboratory"
+ },
+ {
+ "name": "Pai Szu",
+ "pos_x": 114.03125,
+ "pos_y": -18.1875,
+ "pos_z": 65.4375,
+ "stations": "Card Vision,Brust Reach,Clifton Survey"
+ },
+ {
+ "name": "Paijao",
+ "pos_x": -99.78125,
+ "pos_y": -99.5,
+ "pos_z": 59.375,
+ "stations": "Pinto Platform,Leichhardt Depot,Puleston Relay,Barnes Prospect"
+ },
+ {
+ "name": "Paipai",
+ "pos_x": 97.84375,
+ "pos_y": 7.5,
+ "pos_z": -16.84375,
+ "stations": "Griggs Camp"
+ },
+ {
+ "name": "Paiphu",
+ "pos_x": 99.4375,
+ "pos_y": -17.75,
+ "pos_z": 17.875,
+ "stations": "H. G. Wells Refinery,Jernigan Dock,Lichtenberg Enterprise,Gernhardt Vision"
+ },
+ {
+ "name": "Paita",
+ "pos_x": -128.3125,
+ "pos_y": -61.65625,
+ "pos_z": 106.9375,
+ "stations": "Rodrigues Mine"
+ },
+ {
+ "name": "Paitina",
+ "pos_x": 73.71875,
+ "pos_y": 26.0625,
+ "pos_z": 13.78125,
+ "stations": "Musabayev Base"
+ },
+ {
+ "name": "Paitra",
+ "pos_x": 133.8125,
+ "pos_y": 8.1875,
+ "pos_z": -108.59375,
+ "stations": "Puleston Depot"
+ },
+ {
+ "name": "Paiwatec",
+ "pos_x": 56.21875,
+ "pos_y": -159.6875,
+ "pos_z": -15.28125,
+ "stations": "Siddha Legacy"
+ },
+ {
+ "name": "Paiyati",
+ "pos_x": 131.59375,
+ "pos_y": 62.1875,
+ "pos_z": -15.46875,
+ "stations": "Harvey Gateway,Shinn Works,Seega Station,Hilbert Base"
+ },
+ {
+ "name": "Paiyu",
+ "pos_x": 99.875,
+ "pos_y": -82.0625,
+ "pos_z": 132.71875,
+ "stations": "Marcy Platform,Syromyatnikov Terminal,Riess Installation"
+ },
+ {
+ "name": "Paiyungh",
+ "pos_x": -84.84375,
+ "pos_y": -44.4375,
+ "pos_z": -52.78125,
+ "stations": "McMonagle Dock"
+ },
+ {
+ "name": "Pakadji",
+ "pos_x": 64.90625,
+ "pos_y": 13.6875,
+ "pos_z": 121.53125,
+ "stations": "Chamitoff Point"
+ },
+ {
+ "name": "Pakaia",
+ "pos_x": -19.625,
+ "pos_y": -142.15625,
+ "pos_z": 96.40625,
+ "stations": "Schwarzschild Outpost"
+ },
+ {
+ "name": "Palaemon",
+ "pos_x": -78,
+ "pos_y": 20.875,
+ "pos_z": 11.71875,
+ "stations": "Virtanen Gateway,Grover Point"
+ },
+ {
+ "name": "Palanti",
+ "pos_x": -8.375,
+ "pos_y": -131.09375,
+ "pos_z": -92.96875,
+ "stations": "Anderson Orbital,Hernandez Reach"
+ },
+ {
+ "name": "Paledawa",
+ "pos_x": -9.71875,
+ "pos_y": 49.59375,
+ "pos_z": 121.6875,
+ "stations": "Ellern City"
+ },
+ {
+ "name": "Palee",
+ "pos_x": -58.8125,
+ "pos_y": 43.28125,
+ "pos_z": 98.25,
+ "stations": "Thagard Gateway,Buffett Gateway,Bayliss Ring,Marley Horizons"
+ },
+ {
+ "name": "Palekereg",
+ "pos_x": 88.0625,
+ "pos_y": -137.3125,
+ "pos_z": 18.28125,
+ "stations": "Haldeman Terminal,Coulter Gateway,Wagner Enterprise,Land Enterprise"
+ },
+ {
+ "name": "Palengue",
+ "pos_x": 139.65625,
+ "pos_y": -94.84375,
+ "pos_z": -40.65625,
+ "stations": "Wolszczan Dock,Luther Enterprise,Ostrander's Claim,Garden Terminal,Meuron Hub"
+ },
+ {
+ "name": "Paleni",
+ "pos_x": -94.1875,
+ "pos_y": -75,
+ "pos_z": -7.78125,
+ "stations": "Serre Dock"
+ },
+ {
+ "name": "Paletes",
+ "pos_x": -68.25,
+ "pos_y": -30.15625,
+ "pos_z": 77.125,
+ "stations": "Underwood Gateway"
+ },
+ {
+ "name": "Palis",
+ "pos_x": 58.28125,
+ "pos_y": -30.59375,
+ "pos_z": -49.90625,
+ "stations": "Ejeta City,Swanson Terminal"
+ },
+ {
+ "name": "Paliti",
+ "pos_x": -115.8125,
+ "pos_y": -59.625,
+ "pos_z": 48.21875,
+ "stations": "Schmitz Port,Chios Settlement,Svavarsson Park,Baker Forum"
+ },
+ {
+ "name": "Palivik",
+ "pos_x": 92.6875,
+ "pos_y": -40.78125,
+ "pos_z": -98.3125,
+ "stations": "Lowry Depot"
+ },
+ {
+ "name": "Pallaeni",
+ "pos_x": 112.6875,
+ "pos_y": 47.125,
+ "pos_z": 128.4375,
+ "stations": "Bakewell Point,Eyharts Depot"
+ },
+ {
+ "name": "Palliep",
+ "pos_x": 117.46875,
+ "pos_y": -252.90625,
+ "pos_z": -19.6875,
+ "stations": "Gasparis Dock,Watts Depot"
+ },
+ {
+ "name": "Palliyan",
+ "pos_x": 85.0625,
+ "pos_y": 126.21875,
+ "pos_z": -10.71875,
+ "stations": "Rontgen Gateway,Brand Terminal,Gillekens Station,Eisenstein Keep,Coelho Base"
+ },
+ {
+ "name": "Palliyar",
+ "pos_x": -2.125,
+ "pos_y": -109.3125,
+ "pos_z": -22.65625,
+ "stations": "Endate Refinery,Ziewe Survey,Goodricke Prospect,Wright Plant,Ings Plant"
+ },
+ {
+ "name": "Pallu",
+ "pos_x": -140.65625,
+ "pos_y": 59.875,
+ "pos_z": 17.78125,
+ "stations": "Patrick Mines"
+ },
+ {
+ "name": "Palluvumbo",
+ "pos_x": 173.3125,
+ "pos_y": 55.75,
+ "pos_z": -67.5,
+ "stations": "Compton Dock,Wolfe Colony,Wingrove Refinery"
+ },
+ {
+ "name": "Pamenhari",
+ "pos_x": 25,
+ "pos_y": -32.3125,
+ "pos_z": 136.21875,
+ "stations": "Boming Hub,Gordon Gateway,Gernhardt Dock,Aristotle Vision"
+ },
+ {
+ "name": "Pamequi",
+ "pos_x": -61.1875,
+ "pos_y": -81.9375,
+ "pos_z": 108.71875,
+ "stations": "Comino Gateway,Corte-Real's Progress,Carlisle Depot,Henricks City,Leonov Mines"
+ },
+ {
+ "name": "Paminie",
+ "pos_x": 71.625,
+ "pos_y": 0.5625,
+ "pos_z": -84.8125,
+ "stations": "Gaiman Port,Sarmiento de Gamboa Port"
+ },
+ {
+ "name": "Pan",
+ "pos_x": 14.9375,
+ "pos_y": 40.03125,
+ "pos_z": 22.375,
+ "stations": "Xiaoguan Orbital,Neumann Hub"
+ },
+ {
+ "name": "Panara",
+ "pos_x": 81.8125,
+ "pos_y": -141.59375,
+ "pos_z": 139.71875,
+ "stations": "Drake Beacon,Wendelin Port,Coande Hub"
+ },
+ {
+ "name": "Panarion",
+ "pos_x": 36.625,
+ "pos_y": -10.3125,
+ "pos_z": 62.53125,
+ "stations": "Hamilton Gateway,West Hub,Frobisher Orbital,Hume Survey,Bugrov's Claim"
+ },
+ {
+ "name": "Pancegilal",
+ "pos_x": 5.90625,
+ "pos_y": -148.5625,
+ "pos_z": 87.34375,
+ "stations": "Hall Platform,Blish's Progress,Maury Dock"
+ },
+ {
+ "name": "Pancho",
+ "pos_x": -22.09375,
+ "pos_y": 163.875,
+ "pos_z": 30.5625,
+ "stations": "Anthony de la Roche Horizons,Fiennes Point"
+ },
+ {
+ "name": "Panchua",
+ "pos_x": 111.34375,
+ "pos_y": -22.875,
+ "pos_z": -57.28125,
+ "stations": "Tereshkova Hub,Hale Horizons,Covey's Folly"
+ },
+ {
+ "name": "Panci",
+ "pos_x": 74.9375,
+ "pos_y": -55.125,
+ "pos_z": 147.375,
+ "stations": "Druillet Dock,Kaneda Orbital,Ilyushin Vision,Pangborn Plant"
+ },
+ {
+ "name": "Pancienses",
+ "pos_x": 64.4375,
+ "pos_y": -17.5,
+ "pos_z": 43.46875,
+ "stations": "Noli Gateway,Holdstock Enterprise,Mitchell Terminal,Sucharitkul Gateway,Heck Enterprise,Auld Orbital"
+ },
+ {
+ "name": "Pand",
+ "pos_x": -10.03125,
+ "pos_y": -2.40625,
+ "pos_z": 207.84375,
+ "stations": "Sarmiento de Gamboa Port,Raleigh Point"
+ },
+ {
+ "name": "Pandadjara",
+ "pos_x": 112.15625,
+ "pos_y": 109.8125,
+ "pos_z": -85,
+ "stations": "Alpers Colony,Conklin Reach"
+ },
+ {
+ "name": "Pandemonium",
+ "pos_x": 34.15625,
+ "pos_y": -114.03125,
+ "pos_z": 45.96875,
+ "stations": "Siddha Terminal,Friedrich Peters Ring,Hirayama Port,Zaschka Ring"
+ },
+ {
+ "name": "Pandhis",
+ "pos_x": 82.125,
+ "pos_y": 56,
+ "pos_z": -26.09375,
+ "stations": "Karl Diesel City,Cardano Orbital,Land Relay"
+ },
+ {
+ "name": "Pandin",
+ "pos_x": -19.96875,
+ "pos_y": 117.46875,
+ "pos_z": -101.125,
+ "stations": "RenenBellot Retreat,Gabriel Landing"
+ },
+ {
+ "name": "Pandinus",
+ "pos_x": -114.84375,
+ "pos_y": -92.0625,
+ "pos_z": 6.15625,
+ "stations": "Offutt Installation,Bulychev Mines,Saberhagen Station"
+ },
+ {
+ "name": "Pandjuk",
+ "pos_x": 30.4375,
+ "pos_y": 90.25,
+ "pos_z": 38.1875,
+ "stations": "Binder Point,Roberts Reach"
+ },
+ {
+ "name": "Pandra",
+ "pos_x": -41.125,
+ "pos_y": 54.71875,
+ "pos_z": 81.84375,
+ "stations": "Parsons Terminal,Lazarev City,Selye Station,Murdoch Orbital,Galvani Terminal,Descartes Orbital,Schlegel Hub,Amundsen's Progress,Curie Vista"
+ },
+ {
+ "name": "Pang",
+ "pos_x": 121.5625,
+ "pos_y": -18.03125,
+ "pos_z": -87.84375,
+ "stations": "Kazantsev Freeport,Slade Settlement"
+ },
+ {
+ "name": "Pang Guans",
+ "pos_x": -17.1875,
+ "pos_y": 19.6875,
+ "pos_z": 146,
+ "stations": "Pratchett Hub,Hamilton Horizons,Watts Ring,Nagata Station"
+ },
+ {
+ "name": "Panga",
+ "pos_x": 81.9375,
+ "pos_y": -232.65625,
+ "pos_z": 13.1875,
+ "stations": "Galle Settlement,Akers Enterprise,Celebi's Inheritance,Peltier Vision"
+ },
+ {
+ "name": "Panganau",
+ "pos_x": 142.40625,
+ "pos_y": -140.8125,
+ "pos_z": 39.6875,
+ "stations": "Hussenot Ring,Dowie Holdings"
+ },
+ {
+ "name": "Pangerang",
+ "pos_x": 31.34375,
+ "pos_y": 33.15625,
+ "pos_z": 104.65625,
+ "stations": "Oluwafemi Hub,Chamitoff Hub"
+ },
+ {
+ "name": "Pangilagara",
+ "pos_x": 124.1875,
+ "pos_y": -118.96875,
+ "pos_z": 57.8125,
+ "stations": "Tange Vision,Cesar Janssen Bastion,Siodmak Lab"
+ },
+ {
+ "name": "Pangkomo",
+ "pos_x": -104.0625,
+ "pos_y": -11.34375,
+ "pos_z": -131.6875,
+ "stations": "Elwood Dock,Harrison Ring,Tudela Legacy"
+ },
+ {
+ "name": "Pangluya",
+ "pos_x": 27.1875,
+ "pos_y": 41.1875,
+ "pos_z": -16.59375,
+ "stations": "Wang Market,Crampton Port,Morgan Station"
+ },
+ {
+ "name": "Pangondian",
+ "pos_x": -36.96875,
+ "pos_y": 133.21875,
+ "pos_z": -47.53125,
+ "stations": "Alexandria Freeport"
+ },
+ {
+ "name": "Pangwa",
+ "pos_x": 94.96875,
+ "pos_y": 98.71875,
+ "pos_z": 103.5625,
+ "stations": "Tombaugh Relay"
+ },
+ {
+ "name": "Panik",
+ "pos_x": -31.9375,
+ "pos_y": 78.375,
+ "pos_z": 87.15625,
+ "stations": "Bennett City,Crouch Hub,Babcock Station,Smith's Inheritance"
+ },
+ {
+ "name": "Panitollemi",
+ "pos_x": -22,
+ "pos_y": -24.375,
+ "pos_z": 95.1875,
+ "stations": "Papin Installation,Hartlib Platform"
+ },
+ {
+ "name": "Panjabell",
+ "pos_x": -138.09375,
+ "pos_y": -84.28125,
+ "pos_z": 44.9375,
+ "stations": "Agassiz Settlement,Moore Hub,Nemere City,Davidson Dock"
+ },
+ {
+ "name": "Pano",
+ "pos_x": 103,
+ "pos_y": 45.21875,
+ "pos_z": -55.625,
+ "stations": "Aleksandrov Holdings,Kanwar Base"
+ },
+ {
+ "name": "Panoi",
+ "pos_x": -17.75,
+ "pos_y": -9.46875,
+ "pos_z": 60.3125,
+ "stations": "Fairbairn Base,Garan Hub,Garn Settlement"
+ },
+ {
+ "name": "Panomi",
+ "pos_x": -48.4375,
+ "pos_y": 86.15625,
+ "pos_z": 38.46875,
+ "stations": "Lowry Dock,Varley Stop,Fawcett Installation"
+ },
+ {
+ "name": "Panopi",
+ "pos_x": 65.5625,
+ "pos_y": 31.0625,
+ "pos_z": 38.875,
+ "stations": "Smith Plant,Gillekens Holdings"
+ },
+ {
+ "name": "Panorua",
+ "pos_x": 115.78125,
+ "pos_y": 1.59375,
+ "pos_z": 8.0625,
+ "stations": "Oersted Port,Noguchi Terminal,Xiaoguan City,Marconi Orbital,Culpeper Terminal,Scalzi Landing,Stuart Survey,Patrick Dock,Caselberg Town,Searfoss Station,Onizuka Relay"
+ },
+ {
+ "name": "Panossini",
+ "pos_x": 59.53125,
+ "pos_y": -74.1875,
+ "pos_z": 2.65625,
+ "stations": "Adams Penal colony,Fermat Hub,Gottlob Frege Port,Effinger Horizons"
+ },
+ {
+ "name": "Papa",
+ "pos_x": 11.78125,
+ "pos_y": -15.0625,
+ "pos_z": 168.28125,
+ "stations": "Rushd Works,Herjulfsson Settlement"
+ },
+ {
+ "name": "Papai",
+ "pos_x": 108.53125,
+ "pos_y": 83.875,
+ "pos_z": -9,
+ "stations": "Savery Gateway,Noakes Enterprise,Vinci Station"
+ },
+ {
+ "name": "Papako",
+ "pos_x": 141.1875,
+ "pos_y": -218.34375,
+ "pos_z": 124.78125,
+ "stations": "Smirnova Port,Langley Orbital,Goonan Base"
+ },
+ {
+ "name": "Para",
+ "pos_x": -31.53125,
+ "pos_y": 120.1875,
+ "pos_z": -11,
+ "stations": "Russell Colony"
+ },
+ {
+ "name": "Para Indra",
+ "pos_x": -2.15625,
+ "pos_y": 107.34375,
+ "pos_z": -85.9375,
+ "stations": "Pook Orbital,Alexander Orbital,Hand Port,Hoyle Station,Rey Ring"
+ },
+ {
+ "name": "Paraiowal",
+ "pos_x": -78.28125,
+ "pos_y": -123.3125,
+ "pos_z": 23.9375,
+ "stations": "Barentsz Settlement,Yamazaki Survey,Read Retreat"
+ },
+ {
+ "name": "Parakan",
+ "pos_x": 113.625,
+ "pos_y": 64.8125,
+ "pos_z": -89.125,
+ "stations": "Hadfield Vision"
+ },
+ {
+ "name": "Parakana",
+ "pos_x": -65.5,
+ "pos_y": -116.65625,
+ "pos_z": -107.5625,
+ "stations": "Haarsma Horizons,Cook Relay,Kiernan Terminal"
+ },
+ {
+ "name": "Parakara",
+ "pos_x": -100,
+ "pos_y": 54.84375,
+ "pos_z": 81.125,
+ "stations": "O'Brien Terminal,Escobar Vision"
+ },
+ {
+ "name": "Param",
+ "pos_x": -57.84375,
+ "pos_y": 80.375,
+ "pos_z": -31.09375,
+ "stations": "Darboux Base,Temple's Folly"
+ },
+ {
+ "name": "Paramburi",
+ "pos_x": -108.9375,
+ "pos_y": 50.53125,
+ "pos_z": 14.78125,
+ "stations": "Piccard Orbital,Watt-Evans Vision,Kregel Installation"
+ },
+ {
+ "name": "Parana",
+ "pos_x": -118.375,
+ "pos_y": -46.90625,
+ "pos_z": 37.53125,
+ "stations": "Blackman Settlement,Adams Hub"
+ },
+ {
+ "name": "Parapa",
+ "pos_x": 110.40625,
+ "pos_y": 54.84375,
+ "pos_z": -32.84375,
+ "stations": "O'Donnell Terminal,Tevis Station,Paulo da Gama Dock,Bujold Oasis,Kirk Survey"
+ },
+ {
+ "name": "Paras",
+ "pos_x": 56.5,
+ "pos_y": -143.875,
+ "pos_z": 20.75,
+ "stations": "Smoot Station,Wilson Orbital,Barmin Vision"
+ },
+ {
+ "name": "Parasvari",
+ "pos_x": 130.84375,
+ "pos_y": -98.90625,
+ "pos_z": 30.125,
+ "stations": "Lindblad Platform"
+ },
+ {
+ "name": "Paratolit",
+ "pos_x": 81.1875,
+ "pos_y": -150.53125,
+ "pos_z": 118.9375,
+ "stations": "Chertovsky Landing"
+ },
+ {
+ "name": "Paraudika",
+ "pos_x": 49.40625,
+ "pos_y": -22.40625,
+ "pos_z": 126.6875,
+ "stations": "Donaldson Gateway,Tem Station,Mouhot Port,Hottot Station,Resnick Vision"
+ },
+ {
+ "name": "Paray",
+ "pos_x": -95.0625,
+ "pos_y": 65.71875,
+ "pos_z": 116.21875,
+ "stations": "Gooch Survey,Cleave Port"
+ },
+ {
+ "name": "Parcae",
+ "pos_x": -8.125,
+ "pos_y": 55.09375,
+ "pos_z": -17,
+ "stations": "Thiele Relay,Edison Survey,Perry Barracks"
+ },
+ {
+ "name": "Pard",
+ "pos_x": -59.875,
+ "pos_y": -158.53125,
+ "pos_z": -19.3125,
+ "stations": "Oort Station,Geller Beacon"
+ },
+ {
+ "name": "Pardal",
+ "pos_x": -57.21875,
+ "pos_y": 15.4375,
+ "pos_z": 105.78125,
+ "stations": "Gibson Stop"
+ },
+ {
+ "name": "Pardan",
+ "pos_x": 96.65625,
+ "pos_y": -172.5625,
+ "pos_z": 108.1875,
+ "stations": "Potocnik Survey,Keldysh Prospect,Kerimov Holdings,McKee Terminal"
+ },
+ {
+ "name": "Pardh",
+ "pos_x": 123.09375,
+ "pos_y": -206.84375,
+ "pos_z": 51.8125,
+ "stations": "Arnold Dock,Taylor Jr. Relay"
+ },
+ {
+ "name": "Pardhi",
+ "pos_x": 6.1875,
+ "pos_y": -38.28125,
+ "pos_z": 108.40625,
+ "stations": "Vakhmistrov Works,Katzenstein Survey"
+ },
+ {
+ "name": "Pardian",
+ "pos_x": -52.15625,
+ "pos_y": -33.3125,
+ "pos_z": -94.90625,
+ "stations": "Sharma Landing"
+ },
+ {
+ "name": "Pardii",
+ "pos_x": -42.59375,
+ "pos_y": -173.8125,
+ "pos_z": 51.625,
+ "stations": "Laurent Terminal,Brouwer Port,Isherwood Base,Bailly Dock"
+ },
+ {
+ "name": "Pardis",
+ "pos_x": 152.5625,
+ "pos_y": -98.3125,
+ "pos_z": 117.46875,
+ "stations": "Charlois Port,Armero Mines,Extra Hub,Lukyanenko Base,Salak's Folly"
+ },
+ {
+ "name": "Pardok",
+ "pos_x": 122.9375,
+ "pos_y": -174.25,
+ "pos_z": 128.4375,
+ "stations": "Kelly Prospect,Luu Prospect,Dean Relay"
+ },
+ {
+ "name": "Pareco",
+ "pos_x": -77.3125,
+ "pos_y": 12.3125,
+ "pos_z": 21.40625,
+ "stations": "Asire Dock,Garden Ring,Crown Orbital,Webb Station,Phillips Market,Neville Ring"
+ },
+ {
+ "name": "Parecomasci",
+ "pos_x": 161.84375,
+ "pos_y": -65.84375,
+ "pos_z": 59.875,
+ "stations": "Tomita Survey"
+ },
+ {
+ "name": "Parecoyenni",
+ "pos_x": 155.625,
+ "pos_y": -81.375,
+ "pos_z": -24.875,
+ "stations": "Plancius Colony,Kazantsev Beacon,Laming Terminal,Ulloa Platform"
+ },
+ {
+ "name": "Pared",
+ "pos_x": -8.4375,
+ "pos_y": 89.1875,
+ "pos_z": -43.75,
+ "stations": "Reed Station,Elder Hub"
+ },
+ {
+ "name": "Pareda",
+ "pos_x": 58.125,
+ "pos_y": 129.03125,
+ "pos_z": 29.71875,
+ "stations": "Nye Terminal,Moisuc Orbital,Bernard Port,Kirchoff Terminal,Krikalev Hub,Paulo da Gama Keep,Ron Hubbard Holdings"
+ },
+ {
+ "name": "Paredarerme",
+ "pos_x": 83.9375,
+ "pos_y": 8.625,
+ "pos_z": -83.34375,
+ "stations": "McCaffrey Hangar,Sturgeon City,Bombelli Escape"
+ },
+ {
+ "name": "Pareldi",
+ "pos_x": -7.375,
+ "pos_y": 67.28125,
+ "pos_z": 152,
+ "stations": "Sohl Landing,Bao Installation"
+ },
+ {
+ "name": "Parenich",
+ "pos_x": 87.09375,
+ "pos_y": 2.3125,
+ "pos_z": -137.875,
+ "stations": "Harvey Hangar"
+ },
+ {
+ "name": "Parenni",
+ "pos_x": -39.0625,
+ "pos_y": -72.46875,
+ "pos_z": -60.78125,
+ "stations": "Alvares City"
+ },
+ {
+ "name": "Parens",
+ "pos_x": -23.59375,
+ "pos_y": 52.5625,
+ "pos_z": 108.4375,
+ "stations": "Thompson Orbital,Stirling Gateway,Frobenius Silo"
+ },
+ {
+ "name": "Parenses",
+ "pos_x": 91.90625,
+ "pos_y": -24.28125,
+ "pos_z": -22.96875,
+ "stations": "Dobrovolskiy Ring,Fabian Enterprise,Ivanchenkov City,Meade Dock,Wait Colburn Works"
+ },
+ {
+ "name": "Paresa",
+ "pos_x": 86.4375,
+ "pos_y": -197.46875,
+ "pos_z": 28.78125,
+ "stations": "Dyson City,Kahn Orbital,Koishikawa Port,Baker Depot,Knight Base,Alexandrov Vista,Porco Horizons"
+ },
+ {
+ "name": "Paresatsaut",
+ "pos_x": -108.375,
+ "pos_y": 76.21875,
+ "pos_z": -103.625,
+ "stations": "Gordon Enterprise,Waldrop Mines"
+ },
+ {
+ "name": "Parezmia",
+ "pos_x": -202.0625,
+ "pos_y": 50.21875,
+ "pos_z": -47.625,
+ "stations": "Jones Dock"
+ },
+ {
+ "name": "Parhaiya",
+ "pos_x": -91.125,
+ "pos_y": 98.53125,
+ "pos_z": -40.21875,
+ "stations": "Kessel Lab,Kregel Point,Aleksandrov Base,Belyayev Orbital"
+ },
+ {
+ "name": "Parhua",
+ "pos_x": -96.53125,
+ "pos_y": -89.28125,
+ "pos_z": 15.40625,
+ "stations": "Chalker Settlement,Matthews Landing"
+ },
+ {
+ "name": "Parhul",
+ "pos_x": 190.71875,
+ "pos_y": -119.40625,
+ "pos_z": 35.9375,
+ "stations": "Samos Works,Oren Point"
+ },
+ {
+ "name": "Paria",
+ "pos_x": 65.5625,
+ "pos_y": -15.71875,
+ "pos_z": -140.4375,
+ "stations": "Milnor Terminal,Denton Stop,Erdos Landing"
+ },
+ {
+ "name": "Pariabaka",
+ "pos_x": 26.03125,
+ "pos_y": 55.625,
+ "pos_z": -148.34375,
+ "stations": "Rusch City,Tavares Ring,Plucker Vision,Murdoch Vision"
+ },
+ {
+ "name": "Paribeb",
+ "pos_x": -27.3125,
+ "pos_y": 127.90625,
+ "pos_z": -121.0625,
+ "stations": "Bryant Orbital,Gerlache Vision,Hasse Vision"
+ },
+ {
+ "name": "Paribela",
+ "pos_x": -67.9375,
+ "pos_y": 2.4375,
+ "pos_z": 83.65625,
+ "stations": "Ito Hangar"
+ },
+ {
+ "name": "Parinta",
+ "pos_x": -155.65625,
+ "pos_y": 8.71875,
+ "pos_z": -64.875,
+ "stations": "Dukaj Beacon,Linaweaver's Folly"
+ },
+ {
+ "name": "Parja",
+ "pos_x": 18.6875,
+ "pos_y": -67.125,
+ "pos_z": 151.5625,
+ "stations": "Fraknoi Holdings,Wang Platform,Fox Base"
+ },
+ {
+ "name": "Parjanya",
+ "pos_x": -17.65625,
+ "pos_y": 51.59375,
+ "pos_z": 33.375,
+ "stations": "Volterra Enterprise,Gernsback Gateway,Lee Hub,Cavalieri Bastion"
+ },
+ {
+ "name": "Parnabog",
+ "pos_x": 50.15625,
+ "pos_y": 74.125,
+ "pos_z": -71.96875,
+ "stations": "Wyeth Colony,Eddington Landing,King Stop"
+ },
+ {
+ "name": "Parnian",
+ "pos_x": 140.84375,
+ "pos_y": -124.78125,
+ "pos_z": 145,
+ "stations": "Parker's Claim,Maitz Relay"
+ },
+ {
+ "name": "Parnut",
+ "pos_x": -18.75,
+ "pos_y": -33.84375,
+ "pos_z": -57.6875,
+ "stations": "West Point,Meyrink Dock"
+ },
+ {
+ "name": "Parnuteni",
+ "pos_x": -158.28125,
+ "pos_y": 52.96875,
+ "pos_z": 16.53125,
+ "stations": "Stableford Vision,Wilhelm Station,Chorel Settlement,Miller Settlement,Hassanein Holdings"
+ },
+ {
+ "name": "Paroja",
+ "pos_x": 122.1875,
+ "pos_y": 18.96875,
+ "pos_z": -57.75,
+ "stations": "Peterson Camp,Garriott Horizons,Remec Laboratory"
+ },
+ {
+ "name": "Parorans",
+ "pos_x": 101.53125,
+ "pos_y": -203.8125,
+ "pos_z": -57.96875,
+ "stations": "Parsons Orbital,Watson Prospect,Adams Landing,Moran Base"
+ },
+ {
+ "name": "Parovii",
+ "pos_x": -106.03125,
+ "pos_y": -74,
+ "pos_z": 77.71875,
+ "stations": "Borisenko Relay,Smith's Progress"
+ },
+ {
+ "name": "Parowah",
+ "pos_x": -116.71875,
+ "pos_y": 58.5625,
+ "pos_z": 101.15625,
+ "stations": "Lenthall Prospect,McCaffrey Refinery"
+ },
+ {
+ "name": "Pars",
+ "pos_x": 156.96875,
+ "pos_y": -123.3125,
+ "pos_z": -40.15625,
+ "stations": "Schoenherr Settlement,Ikeya Horizons,Lalande Relay,Dalmas Reach"
+ },
+ {
+ "name": "Parsaka",
+ "pos_x": 76.4375,
+ "pos_y": 79.53125,
+ "pos_z": 55.5,
+ "stations": "MacLean Arena"
+ },
+ {
+ "name": "Parsi",
+ "pos_x": -31.78125,
+ "pos_y": -133.15625,
+ "pos_z": 11.8125,
+ "stations": "Helffrich Dock,Gould Settlement,Froude Prospect,Kirk Works"
+ },
+ {
+ "name": "Parta",
+ "pos_x": 87,
+ "pos_y": 17.03125,
+ "pos_z": -56.65625,
+ "stations": "Shargin Port,Delbruck Enterprise,Thagard Mine,Strekalov Enterprise,Forstchen Mine"
+ },
+ {
+ "name": "Partha",
+ "pos_x": -6.46875,
+ "pos_y": -4.34375,
+ "pos_z": 111.25,
+ "stations": "Gresley Survey,Ferguson Silo"
+ },
+ {
+ "name": "Parthians",
+ "pos_x": -56.21875,
+ "pos_y": -89.09375,
+ "pos_z": 92.625,
+ "stations": "Houtman Orbital,Tudela Platform,McMullen's Inheritance,Lowry Vision,Scheerbart Gateway,Peirce Ring"
+ },
+ {
+ "name": "Parthini",
+ "pos_x": 114.40625,
+ "pos_y": 23.125,
+ "pos_z": -96.71875,
+ "stations": "Auer Gateway"
+ },
+ {
+ "name": "Parti",
+ "pos_x": 127.90625,
+ "pos_y": 44.25,
+ "pos_z": 30.28125,
+ "stations": "Brash Landing,Stefanyshyn-Piper Terminal,Wallace Base"
+ },
+ {
+ "name": "Partii",
+ "pos_x": 41.5625,
+ "pos_y": -196.90625,
+ "pos_z": -64.21875,
+ "stations": "Cheranovsky City,Sitterly Terminal,Fairbairn Hub"
+ },
+ {
+ "name": "Paru",
+ "pos_x": 103.15625,
+ "pos_y": -89.6875,
+ "pos_z": 107.0625,
+ "stations": "Courvoisier Port,Galouye Base,Keeler Mines"
+ },
+ {
+ "name": "Parulang",
+ "pos_x": 174.75,
+ "pos_y": -150.09375,
+ "pos_z": 19.90625,
+ "stations": "Schoening Terminal"
+ },
+ {
+ "name": "Parun",
+ "pos_x": -38.09375,
+ "pos_y": -123.6875,
+ "pos_z": -60.0625,
+ "stations": "Underwood Port,Foucault Gateway,Shepherd Colony,Pippin Enterprise"
+ },
+ {
+ "name": "Paruna",
+ "pos_x": -96.84375,
+ "pos_y": 111.75,
+ "pos_z": 16.25,
+ "stations": "Hand Colony,Farouk Horizons,Gibbs Port,Gernhardt Installation"
+ },
+ {
+ "name": "Parutis",
+ "pos_x": 0.71875,
+ "pos_y": 10.71875,
+ "pos_z": 33.28125,
+ "stations": "Evans Port,Parmitano Station,Schweickart City,Teng-hui Hub,Shuttleworth Terminal,Menzies Penal colony,Nelson Base"
+ },
+ {
+ "name": "Pastara",
+ "pos_x": 17.9375,
+ "pos_y": -149.5,
+ "pos_z": -20.625,
+ "stations": "Shiner Port,Matthews Legacy,Forfait City,Broderick Terminal,Argelander Enterprise,Simak Works,Peebles Depot"
+ },
+ {
+ "name": "Pastii",
+ "pos_x": -57.59375,
+ "pos_y": -78.96875,
+ "pos_z": 124.03125,
+ "stations": "Simmons Enterprise"
+ },
+ {
+ "name": "Patabracoc",
+ "pos_x": 70.28125,
+ "pos_y": -93.15625,
+ "pos_z": 124.1875,
+ "stations": "Crossfield Plant,Thome Orbital,Dietz Enterprise,Charlier Landing,Riess Hub"
+ },
+ {
+ "name": "Pataheimr",
+ "pos_x": -98.90625,
+ "pos_y": -15.78125,
+ "pos_z": 64.375,
+ "stations": "Arnold City,Good Station,Thornycroft's Claim,Birdseye Port,Kavandi Port,Banks Holdings,Elwood Prospect,Rangarajan Bastion"
+ },
+ {
+ "name": "Pataka",
+ "pos_x": 96.625,
+ "pos_y": 36.46875,
+ "pos_z": 10,
+ "stations": "Favier Landing,Yegorov Colony,Tito Base,Jett Hub"
+ },
+ {
+ "name": "Patakaka",
+ "pos_x": 77,
+ "pos_y": 140.59375,
+ "pos_z": -17.3125,
+ "stations": "Brunton Hub,Beregovoi Platform,Pasteur City,Vinge Enterprise"
+ },
+ {
+ "name": "Patal",
+ "pos_x": -1.3125,
+ "pos_y": -117.71875,
+ "pos_z": -52.9375,
+ "stations": "Kaku Landing"
+ },
+ {
+ "name": "Patanawi",
+ "pos_x": 70.5625,
+ "pos_y": 35.21875,
+ "pos_z": -88.46875,
+ "stations": "Hyecho Station,Roddenberry Gateway,Haber Hub,Mitchell Dock,Cixin Dock"
+ },
+ {
+ "name": "Patani",
+ "pos_x": 48.09375,
+ "pos_y": 90.625,
+ "pos_z": -19.4375,
+ "stations": "Smith Ring,Cseszneky Dock,Reisman Keep,Borlaug Terminal,Nielsen's Folly"
+ },
+ {
+ "name": "Patayony",
+ "pos_x": 34.59375,
+ "pos_y": -13,
+ "pos_z": 119.59375,
+ "stations": "Zetford Hub,Crown Bastion,Lewitt Gateway"
+ },
+ {
+ "name": "Pate",
+ "pos_x": -74.40625,
+ "pos_y": -83.28125,
+ "pos_z": -118.28125,
+ "stations": "Mitropoulos Station"
+ },
+ {
+ "name": "Path",
+ "pos_x": -80.0625,
+ "pos_y": -47.5625,
+ "pos_z": 115.125,
+ "stations": "Curie Mines,Carpenter Port,Agnesi Plant"
+ },
+ {
+ "name": "Patha",
+ "pos_x": -65.8125,
+ "pos_y": 104.59375,
+ "pos_z": 46.53125,
+ "stations": "Gell-Mann Terminal,Bose Beacon"
+ },
+ {
+ "name": "Pathamon",
+ "pos_x": -77.96875,
+ "pos_y": -137.75,
+ "pos_z": -88.6875,
+ "stations": "Klein Survey,Sohl Port,Cavalieri Landing,Banks Prospect,Kube-McDowell Horizons"
+ },
+ {
+ "name": "Pathan",
+ "pos_x": -71.8125,
+ "pos_y": -51.5,
+ "pos_z": -48.90625,
+ "stations": "Smith Port,McMonagle Port,Marshall Ring,Hertz Station,Carr City,MacVicar Keep,Conway Vision"
+ },
+ {
+ "name": "Pathokula",
+ "pos_x": 18.625,
+ "pos_y": 157.84375,
+ "pos_z": -97.53125,
+ "stations": "Norman Dock,Remec Barracks"
+ },
+ {
+ "name": "Patocuda",
+ "pos_x": -81.9375,
+ "pos_y": -100.46875,
+ "pos_z": 18.78125,
+ "stations": "Coney Gateway,Bentham Hub,Auer Station,Fernao do Po Port,Bao Orbital,Noon Enterprise,Fadlan's Claim,Francisco de Almeida Vision,Shatner's Folly,Brule Relay,Hausdorff Hub,Morrow's Inheritance,Levi-Civita Base"
+ },
+ {
+ "name": "Patollo",
+ "pos_x": -66.46875,
+ "pos_y": 91.0625,
+ "pos_z": -59.125,
+ "stations": "Balmer Prospect,Russell Mine"
+ },
+ {
+ "name": "Patollu",
+ "pos_x": -54.65625,
+ "pos_y": -131.96875,
+ "pos_z": -93.09375,
+ "stations": "Oliver's Progress,Krupkat Mine,Garrett Arsenal"
+ },
+ {
+ "name": "Patora",
+ "pos_x": -100.28125,
+ "pos_y": 96.0625,
+ "pos_z": -100.21875,
+ "stations": "Ham Gateway,Bernoulli Terminal,McKay Dock,Griffin Horizons"
+ },
+ {
+ "name": "Patosata",
+ "pos_x": 53.53125,
+ "pos_y": -75.0625,
+ "pos_z": 112.34375,
+ "stations": "Parker Hub,Gustav Sporer Settlement,Fuller Installation,Binney Orbital"
+ },
+ {
+ "name": "Patrudihe",
+ "pos_x": -27.84375,
+ "pos_y": 97.21875,
+ "pos_z": -35.84375,
+ "stations": "Hambly Port"
+ },
+ {
+ "name": "Patrug",
+ "pos_x": -22.84375,
+ "pos_y": -117.53125,
+ "pos_z": 88.9375,
+ "stations": "Xuesen Station"
+ },
+ {
+ "name": "Pauahtun",
+ "pos_x": -43.5625,
+ "pos_y": -24.4375,
+ "pos_z": 117.59375,
+ "stations": "Andrews Gateway,Utley Silo,Szebehely Horizons,MacKellar Landing"
+ },
+ {
+ "name": "Pauang",
+ "pos_x": -97.65625,
+ "pos_y": -92.8125,
+ "pos_z": -120.40625,
+ "stations": "Killough City,Zettel City,Snodgrass Station,Fearn Colony"
+ },
+ {
+ "name": "Pauatunus",
+ "pos_x": 83.3125,
+ "pos_y": 6.625,
+ "pos_z": -48.09375,
+ "stations": "Asimov Landing,Clark Platform,Garcia Landing,Alvares Horizons"
+ },
+ {
+ "name": "Paucatec",
+ "pos_x": -33.625,
+ "pos_y": 65.15625,
+ "pos_z": 101.125,
+ "stations": "Clauss Refinery,Markov Landing"
+ },
+ {
+ "name": "Paucates",
+ "pos_x": 130.125,
+ "pos_y": -75.15625,
+ "pos_z": -37.84375,
+ "stations": "Deutsch Orbital,Amundsen Bastion,Bretnor Vision"
+ },
+ {
+ "name": "Paucuman",
+ "pos_x": 55.28125,
+ "pos_y": -163.625,
+ "pos_z": -82.1875,
+ "stations": "Littrow Vision,Beriev Lab,Wright Orbital"
+ },
+ {
+ "name": "Pauish",
+ "pos_x": 60.21875,
+ "pos_y": -202.625,
+ "pos_z": -21.125,
+ "stations": "Wilson Base,Giger Mine"
+ },
+ {
+ "name": "Pauishana",
+ "pos_x": -26.3125,
+ "pos_y": 145.34375,
+ "pos_z": 47,
+ "stations": "Conklin Enterprise,Lee Mine"
+ },
+ {
+ "name": "Paul-Friedrichs Star",
+ "pos_x": -48.15625,
+ "pos_y": 34.3125,
+ "pos_z": 8.78125,
+ "stations": "Paul-Friedrichs Expedition Base Camp"
+ },
+ {
+ "name": "Pawi",
+ "pos_x": 113.6875,
+ "pos_y": -162.5625,
+ "pos_z": 124.25,
+ "stations": "Dowty Dock,Weaver Vision,McMullen Vista,Belyavsky Beacon"
+ },
+ {
+ "name": "Pawilatal",
+ "pos_x": -7.875,
+ "pos_y": 83.1875,
+ "pos_z": 97.21875,
+ "stations": "Stebler Survey,Shiras Keep"
+ },
+ {
+ "name": "Payan",
+ "pos_x": 95.1875,
+ "pos_y": 63.9375,
+ "pos_z": -97.53125,
+ "stations": "Elwood Gateway,Levy Hub,Popov Colony"
+ },
+ {
+ "name": "Payana",
+ "pos_x": 58.90625,
+ "pos_y": 100.3125,
+ "pos_z": -31.25,
+ "stations": "Baker Market"
+ },
+ {
+ "name": "Payatamu",
+ "pos_x": -148.65625,
+ "pos_y": 45.65625,
+ "pos_z": -38.9375,
+ "stations": "Bushnell City"
+ },
+ {
+ "name": "Payayan",
+ "pos_x": -12.125,
+ "pos_y": 56.59375,
+ "pos_z": 9.1875,
+ "stations": "Stafford Hub,Phillifent Enterprise,Reed Relay,Pinto City,Bierce Gateway,Gibson City,Russell Station,O'Leary Relay,Finch Ring"
+ },
+ {
+ "name": "Peacock",
+ "pos_x": 47.0625,
+ "pos_y": -104.46875,
+ "pos_z": 137.28125,
+ "stations": "Phillips Dock,Dickson Base,Land Barracks"
+ },
+ {
+ "name": "Pech",
+ "pos_x": -81.5,
+ "pos_y": 42.03125,
+ "pos_z": 130.53125,
+ "stations": "Brash Dock,Vogel Port"
+ },
+ {
+ "name": "Pech Bean",
+ "pos_x": 80.09375,
+ "pos_y": -163.59375,
+ "pos_z": -83.8125,
+ "stations": "Wood Orbital,Egan Relay"
+ },
+ {
+ "name": "Peche",
+ "pos_x": 60.8125,
+ "pos_y": -54.6875,
+ "pos_z": 79.875,
+ "stations": "Brady Holdings,Pavlov Station,Lindsey Dock,Stasheff Landing"
+ },
+ {
+ "name": "Pechs",
+ "pos_x": -139.3125,
+ "pos_y": -23.5625,
+ "pos_z": -24.0625,
+ "stations": "Sommerfeld Dock,Meikle City,Ivins Station,Dupuy de Lome Barracks"
+ },
+ {
+ "name": "Pechua",
+ "pos_x": 62.6875,
+ "pos_y": 35.28125,
+ "pos_z": -80.71875,
+ "stations": "Payson Relay,Magnus Survey"
+ },
+ {
+ "name": "Peckollates",
+ "pos_x": -123.96875,
+ "pos_y": -81,
+ "pos_z": 20.90625,
+ "stations": "Clayton Station,Obruchev Survey,Bacigalupi Works,Bottego Orbital"
+ },
+ {
+ "name": "Peckollerci",
+ "pos_x": -36.125,
+ "pos_y": 99.09375,
+ "pos_z": 14.78125,
+ "stations": "Minkowski Orbital,McDaniel Beacon,Zamyatin's Inheritance,Fernao do Po Horizons"
+ },
+ {
+ "name": "Peckolong",
+ "pos_x": 26.875,
+ "pos_y": 2.6875,
+ "pos_z": 101.96875,
+ "stations": "Eddington Landing,Khayyam Colony,Alexeyev Settlement"
+ },
+ {
+ "name": "Peckolsumij",
+ "pos_x": -73.21875,
+ "pos_y": 108.125,
+ "pos_z": -7.1875,
+ "stations": "Zamyatin Co-operative,Nikitin Co-operative,Miklouho-Maclay City,Pike Hub"
+ },
+ {
+ "name": "Pegasi Sector AB-N b7-8",
+ "pos_x": -151.75,
+ "pos_y": -71.375,
+ "pos_z": 54.375,
+ "stations": "Paez Landing"
+ },
+ {
+ "name": "Pegasi Sector BL-X c1-25",
+ "pos_x": -157.09375,
+ "pos_y": -11.21875,
+ "pos_z": -62.125,
+ "stations": "Asaro Installation,Neff's Folly,Staden Depot"
+ },
+ {
+ "name": "Pegasi Sector BQ-Y d100",
+ "pos_x": -160.3125,
+ "pos_y": -81.09375,
+ "pos_z": -68.6875,
+ "stations": "Borel Holdings"
+ },
+ {
+ "name": "Pegasi Sector BQ-Y d72",
+ "pos_x": -166,
+ "pos_y": -68.25,
+ "pos_z": -57.34375,
+ "stations": "Walker Point"
+ },
+ {
+ "name": "Pegasi Sector BQ-Y d75",
+ "pos_x": -173.9375,
+ "pos_y": -32.96875,
+ "pos_z": -36.90625,
+ "stations": "Conti Landing"
+ },
+ {
+ "name": "Pegasi Sector BQ-Y d92",
+ "pos_x": -163.875,
+ "pos_y": -49.65625,
+ "pos_z": -67.0625,
+ "stations": "McMullen Settlement,Narbeth Survey"
+ },
+ {
+ "name": "Pegasi Sector BQ-Y d94",
+ "pos_x": -172.5,
+ "pos_y": -73.1875,
+ "pos_z": -53.59375,
+ "stations": "Huxley Prison,Bering's Inheritance"
+ },
+ {
+ "name": "Pegasi Sector BV-X b1-7",
+ "pos_x": -135.875,
+ "pos_y": -63.125,
+ "pos_z": -69.125,
+ "stations": "Pordenone Landing"
+ },
+ {
+ "name": "Pegasi Sector BV-X b1-8",
+ "pos_x": -145,
+ "pos_y": -50.0625,
+ "pos_z": -75.4375,
+ "stations": "Brin Beacon"
+ },
+ {
+ "name": "Pegasi Sector CQ-Y d101",
+ "pos_x": -103.3125,
+ "pos_y": -72,
+ "pos_z": -55.25,
+ "stations": "Stanier's Progress"
+ },
+ {
+ "name": "Pegasi Sector CQ-Y d106",
+ "pos_x": -138.28125,
+ "pos_y": -84.03125,
+ "pos_z": -67.90625,
+ "stations": "Pavlou Terminal,Harper Reach"
+ },
+ {
+ "name": "Pegasi Sector CR-S a5-3",
+ "pos_x": -149.1875,
+ "pos_y": -25.0625,
+ "pos_z": -58.875,
+ "stations": "Bruce Hub"
+ },
+ {
+ "name": "Pegasi Sector CV-Y c22",
+ "pos_x": -144.71875,
+ "pos_y": -97.625,
+ "pos_z": -67.84375,
+ "stations": "Ellern's Inheritance"
+ },
+ {
+ "name": "Pegasi Sector DB-X d1-72",
+ "pos_x": -173.375,
+ "pos_y": -23.46875,
+ "pos_z": 38.25,
+ "stations": "Shaara Base"
+ },
+ {
+ "name": "Pegasi Sector DW-M b7-2",
+ "pos_x": -130.1875,
+ "pos_y": -89.8125,
+ "pos_z": 41.5,
+ "stations": "Cogswell Horizons"
+ },
+ {
+ "name": "Pegasi Sector EL-Y d54",
+ "pos_x": -131.96875,
+ "pos_y": -106.78125,
+ "pos_z": -48.8125,
+ "stations": "Mitchison Arsenal,Robinson Terminal"
+ },
+ {
+ "name": "Pegasi Sector EL-Y d96",
+ "pos_x": -127.3125,
+ "pos_y": -141.34375,
+ "pos_z": -56.6875,
+ "stations": "Luiken Installation,Shalatula Terminal"
+ },
+ {
+ "name": "Pegasi Sector EM-S a5-3",
+ "pos_x": -149.40625,
+ "pos_y": -44.28125,
+ "pos_z": -55.21875,
+ "stations": "Gresh Base"
+ },
+ {
+ "name": "Pegasi Sector EW-M b7-2",
+ "pos_x": -124.46875,
+ "pos_y": -104.75,
+ "pos_z": 38.34375,
+ "stations": "Levy Horizons,Fraser's Progress"
+ },
+ {
+ "name": "Pegasi Sector FB-X c1-4",
+ "pos_x": -171.15625,
+ "pos_y": -78.125,
+ "pos_z": -25.15625,
+ "stations": "Morgan Base"
+ },
+ {
+ "name": "Pegasi Sector FD-G a12-2",
+ "pos_x": -162.875,
+ "pos_y": -41.21875,
+ "pos_z": 6.46875,
+ "stations": "Larson Enterprise"
+ },
+ {
+ "name": "Pegasi Sector FL-X b1-2",
+ "pos_x": -144.8125,
+ "pos_y": -92.9375,
+ "pos_z": -70.90625,
+ "stations": "Cowper Enterprise,Dias Landing"
+ },
+ {
+ "name": "Pegasi Sector FL-X b1-3",
+ "pos_x": -140.53125,
+ "pos_y": -86.53125,
+ "pos_z": -75.8125,
+ "stations": "Cartan Depot"
+ },
+ {
+ "name": "Pegasi Sector FR-U b3-9",
+ "pos_x": -138.0625,
+ "pos_y": -19.25,
+ "pos_z": -37.1875,
+ "stations": "Sterling Horizons,Chasles Penal colony,King's Folly"
+ },
+ {
+ "name": "Pegasi Sector FR-V c2-12",
+ "pos_x": -161.03125,
+ "pos_y": -20.21875,
+ "pos_z": -1.46875,
+ "stations": "Warner Vision"
+ },
+ {
+ "name": "Pegasi Sector FR-V c2-23",
+ "pos_x": -176.6875,
+ "pos_y": -0.625,
+ "pos_z": -21.375,
+ "stations": "Houtman's Folly,Griffith Depot"
+ },
+ {
+ "name": "Pegasi Sector FR-V c2-25",
+ "pos_x": -184.3125,
+ "pos_y": -3.25,
+ "pos_z": -24.375,
+ "stations": "Knight Base,Emshwiller Horizons"
+ },
+ {
+ "name": "Pegasi Sector FR-V c2-4",
+ "pos_x": -163.8125,
+ "pos_y": 4.25,
+ "pos_z": -11.1875,
+ "stations": "Cauchy Settlement"
+ },
+ {
+ "name": "Pegasi Sector FR-V c2-7",
+ "pos_x": -173.9375,
+ "pos_y": -11.15625,
+ "pos_z": -5.40625,
+ "stations": "Garcia Barracks"
+ },
+ {
+ "name": "Pegasi Sector FW-W d1-111",
+ "pos_x": -155.59375,
+ "pos_y": -71.25,
+ "pos_z": -20.59375,
+ "stations": "Atkov's Progress"
+ },
+ {
+ "name": "Pegasi Sector FW-W d1-116",
+ "pos_x": -188,
+ "pos_y": -87.625,
+ "pos_z": 46.4375,
+ "stations": "Scheerbart Beacon"
+ },
+ {
+ "name": "Pegasi Sector FW-W d1-59",
+ "pos_x": -159.59375,
+ "pos_y": -77.71875,
+ "pos_z": 10.03125,
+ "stations": "Humphreys Settlement,Alvarado Plant"
+ },
+ {
+ "name": "Pegasi Sector GB-X c1-8",
+ "pos_x": -135.15625,
+ "pos_y": -90.03125,
+ "pos_z": -48.84375,
+ "stations": "Polyakov's Claim,Due Barracks"
+ },
+ {
+ "name": "Pegasi Sector GR-M b7-3",
+ "pos_x": -116.125,
+ "pos_y": -120.25,
+ "pos_z": 36.65625,
+ "stations": "Pale Horse Industrial Complex"
+ },
+ {
+ "name": "Pegasi Sector GR-V c2-30",
+ "pos_x": -142.21875,
+ "pos_y": -24.15625,
+ "pos_z": 1.90625,
+ "stations": "Crown Installation,Filter Terminal,Semeonis Vision"
+ },
+ {
+ "name": "Pegasi Sector GW-W d1-115",
+ "pos_x": -143.6875,
+ "pos_y": -83.3125,
+ "pos_z": -21.375,
+ "stations": "Morris Plant,Mieville Works"
+ },
+ {
+ "name": "Pegasi Sector HC-L b8-6",
+ "pos_x": -141.15625,
+ "pos_y": -104.9375,
+ "pos_z": 74.03125,
+ "stations": "Oliver Relay"
+ },
+ {
+ "name": "Pegasi Sector HM-V c2-16",
+ "pos_x": -160.5,
+ "pos_y": -29.125,
+ "pos_z": -7.5625,
+ "stations": "Gerrold Vision"
+ },
+ {
+ "name": "Pegasi Sector HW-V b2-0",
+ "pos_x": -142.1875,
+ "pos_y": -82.25,
+ "pos_z": -45.96875,
+ "stations": "Foale's Claim"
+ },
+ {
+ "name": "Pegasi Sector IG-X b1-1",
+ "pos_x": -106.8125,
+ "pos_y": -124.90625,
+ "pos_z": -65.3125,
+ "stations": "Bulmer Point"
+ },
+ {
+ "name": "Pegasi Sector IR-W d1-72",
+ "pos_x": -104.09375,
+ "pos_y": -116.8125,
+ "pos_z": 39.0625,
+ "stations": "Pinto Enterprise"
+ },
+ {
+ "name": "Pegasi Sector IR-W d1-90",
+ "pos_x": -122.8125,
+ "pos_y": -110.65625,
+ "pos_z": -16.9375,
+ "stations": "Aldiss Terminal,Macomb Point"
+ },
+ {
+ "name": "Pegasi Sector JX-S b4-9",
+ "pos_x": -136.9375,
+ "pos_y": -9.78125,
+ "pos_z": -6.90625,
+ "stations": "Birkhoff Beacon,Powell Plant,Wiener Installation"
+ },
+ {
+ "name": "Pegasi Sector KJ-E a13-0",
+ "pos_x": -152.21875,
+ "pos_y": -44.0625,
+ "pos_z": 17.90625,
+ "stations": "Brooks Enterprise"
+ },
+ {
+ "name": "Pegasi Sector LS-T c3-16",
+ "pos_x": -178.34375,
+ "pos_y": -48.21875,
+ "pos_z": 26.4375,
+ "stations": "Varley Beacon"
+ },
+ {
+ "name": "Pegasi Sector MC-V c2-12",
+ "pos_x": -136.9375,
+ "pos_y": -108.625,
+ "pos_z": 14.5,
+ "stations": "Shipton Enterprise,Bachman Enterprise"
+ },
+ {
+ "name": "Pegasi Sector MC-V c2-7",
+ "pos_x": -118.6875,
+ "pos_y": -130.28125,
+ "pos_z": -15.15625,
+ "stations": "Lloyd Prospect,Slonczewski Depot"
+ },
+ {
+ "name": "Pegasi Sector MD-R b5-3",
+ "pos_x": -160.09375,
+ "pos_y": -20.125,
+ "pos_z": 11.25,
+ "stations": "Smith Vista"
+ },
+ {
+ "name": "Pegasi Sector MN-S b4-5",
+ "pos_x": -154.375,
+ "pos_y": -45.375,
+ "pos_z": -15.9375,
+ "stations": "Jordan Base"
+ },
+ {
+ "name": "Pegasi Sector NN-T c3-11",
+ "pos_x": -161.78125,
+ "pos_y": -103.84375,
+ "pos_z": 34.0625,
+ "stations": "Eskridge Barracks"
+ },
+ {
+ "name": "Pegasi Sector NN-T c3-18",
+ "pos_x": -153.3125,
+ "pos_y": -65.125,
+ "pos_z": 54.9375,
+ "stations": "Weierstrass Bastion"
+ },
+ {
+ "name": "Pegasi Sector NN-T c3-9",
+ "pos_x": -156.53125,
+ "pos_y": -99.9375,
+ "pos_z": 32.8125,
+ "stations": "Sarmiento de Gamboa Installation,Brooks Relay"
+ },
+ {
+ "name": "Pegasi Sector OX-T b3-0",
+ "pos_x": -114.4375,
+ "pos_y": -95.28125,
+ "pos_z": -42.625,
+ "stations": "Rose Base"
+ },
+ {
+ "name": "Pegasi Sector OX-T b3-3",
+ "pos_x": -114.125,
+ "pos_y": -104.03125,
+ "pos_z": -29.9375,
+ "stations": "al-Khowarizmi Mines,Pangborn Base"
+ },
+ {
+ "name": "Pegasi Sector PH-V b2-0",
+ "pos_x": -104.71875,
+ "pos_y": -131.125,
+ "pos_z": -56.15625,
+ "stations": "Henize's Progress"
+ },
+ {
+ "name": "Pegasi Sector PX-T b3-5",
+ "pos_x": -92.9375,
+ "pos_y": -93.46875,
+ "pos_z": -30.9375,
+ "stations": "Shaw Base"
+ },
+ {
+ "name": "Pegasi Sector PX-U c2-4",
+ "pos_x": -102.90625,
+ "pos_y": -155.03125,
+ "pos_z": 2.34375,
+ "stations": "Crown Enterprise"
+ },
+ {
+ "name": "Pegasi Sector PY-R c4-12",
+ "pos_x": -166.9375,
+ "pos_y": -58.28125,
+ "pos_z": 70.03125,
+ "stations": "Witt Survey"
+ },
+ {
+ "name": "Pegasi Sector QJ-P b6-6",
+ "pos_x": -159.4375,
+ "pos_y": -11.0625,
+ "pos_z": 22.40625,
+ "stations": "Macgregor Prospect"
+ },
+ {
+ "name": "Pegasi Sector RD-S b4-6",
+ "pos_x": -132.78125,
+ "pos_y": -101.78125,
+ "pos_z": -23.65625,
+ "stations": "Fox Landing,Kirtley Prospect"
+ },
+ {
+ "name": "Pegasi Sector TY-R b4-0",
+ "pos_x": -141.71875,
+ "pos_y": -105.25,
+ "pos_z": -10.34375,
+ "stations": "Sturgeon Vision"
+ },
+ {
+ "name": "Pegasi Sector UZ-O b6-5",
+ "pos_x": -156.1875,
+ "pos_y": -64.40625,
+ "pos_z": 22.65625,
+ "stations": "Frobenius Silo,Napier Landing"
+ },
+ {
+ "name": "Pegasi Sector VJ-Q b5-1",
+ "pos_x": -129.5,
+ "pos_y": -98.53125,
+ "pos_z": 8.8125,
+ "stations": "Carver Horizons"
+ },
+ {
+ "name": "Pegasi Sector VO-Q b5-3",
+ "pos_x": -98.65625,
+ "pos_y": -83.25,
+ "pos_z": -4.40625,
+ "stations": "Mallett's Progress,Vasquez de Coronado Depot,Ferguson Enterprise"
+ },
+ {
+ "name": "Pegasi Sector XE-Q b5-0",
+ "pos_x": -139.375,
+ "pos_y": -122.5625,
+ "pos_z": -3.6875,
+ "stations": "Lee Point"
+ },
+ {
+ "name": "Pegasi Sector XO-Z b2",
+ "pos_x": -141.40625,
+ "pos_y": -57.125,
+ "pos_z": -95.15625,
+ "stations": "Harding Works"
+ },
+ {
+ "name": "Pegasi Sector XU-O b6-2",
+ "pos_x": -133.59375,
+ "pos_y": -81.78125,
+ "pos_z": 17.8125,
+ "stations": "Wingrove Relay,Zebrowski Beacon"
+ },
+ {
+ "name": "Pegasi Sector XU-O b6-3",
+ "pos_x": -130.875,
+ "pos_y": -80.625,
+ "pos_z": 16.03125,
+ "stations": "Terry's Progress"
+ },
+ {
+ "name": "Pegasi Sector YE-Q b5-4",
+ "pos_x": -122.9375,
+ "pos_y": -123.25,
+ "pos_z": 13.4375,
+ "stations": "Grant Terminal"
+ },
+ {
+ "name": "Pehuti",
+ "pos_x": -23.625,
+ "pos_y": 79.53125,
+ "pos_z": -140.03125,
+ "stations": "Ali Point,Morukov Platform"
+ },
+ {
+ "name": "Pekoe",
+ "pos_x": -9485.75,
+ "pos_y": -887.96875,
+ "pos_z": 19804.21875,
+ "stations": "Jonas Station"
+ },
+ {
+ "name": "Pel",
+ "pos_x": 80.40625,
+ "pos_y": -218.03125,
+ "pos_z": 0.21875,
+ "stations": "Opik Hub,Duque Mines,Schumacher Settlement"
+ },
+ {
+ "name": "Pelin",
+ "pos_x": 52.40625,
+ "pos_y": 57.125,
+ "pos_z": 42.84375,
+ "stations": "Crouch Dock,Jernigan Refinery"
+ },
+ {
+ "name": "Pell",
+ "pos_x": -0.6875,
+ "pos_y": -54.03125,
+ "pos_z": 85.625,
+ "stations": "Shaara Prospect,Huxley Dock,Luiken Hub"
+ },
+ {
+ "name": "Pella",
+ "pos_x": 69.8125,
+ "pos_y": -139.53125,
+ "pos_z": -56.15625,
+ "stations": "Pollas Base,Arzachel Holdings,Hoffmeister Oasis"
+ },
+ {
+ "name": "Pellang",
+ "pos_x": 13.375,
+ "pos_y": -57.46875,
+ "pos_z": 70.9375,
+ "stations": "Ising Landing,Flynn's Progress,Chargaff Mines"
+ },
+ {
+ "name": "Pelleru",
+ "pos_x": 143.125,
+ "pos_y": -79.09375,
+ "pos_z": -17.96875,
+ "stations": "Spassky Hub,Heinkel Horizons,Morgan Vision"
+ },
+ {
+ "name": "Pemede",
+ "pos_x": -88.4375,
+ "pos_y": 25.46875,
+ "pos_z": -26.15625,
+ "stations": "Leavitt Orbital,Gurragchaa Hub,Levinson Vista,Gidzenko Terminal,Wankel Plant"
+ },
+ {
+ "name": "Pemeno",
+ "pos_x": 27.71875,
+ "pos_y": -178.25,
+ "pos_z": -58.5625,
+ "stations": "Graham Beacon"
+ },
+ {
+ "name": "Pemeowa",
+ "pos_x": 16.0625,
+ "pos_y": -106.4375,
+ "pos_z": 7.34375,
+ "stations": "Burke Beacon"
+ },
+ {
+ "name": "Pemepatung",
+ "pos_x": 75.46875,
+ "pos_y": -69.4375,
+ "pos_z": -76.9375,
+ "stations": "Makeev Orbital,Salgari Vision,Jakes Vision"
+ },
+ {
+ "name": "Pemez",
+ "pos_x": -76.0625,
+ "pos_y": -124.375,
+ "pos_z": 6.75,
+ "stations": "Andree Asylum"
+ },
+ {
+ "name": "Pemoeri",
+ "pos_x": 68.71875,
+ "pos_y": 32.75,
+ "pos_z": 41.03125,
+ "stations": "Lousma Port,al-Din Landing,Weber Barracks"
+ },
+ {
+ "name": "Pemoten",
+ "pos_x": -61.0625,
+ "pos_y": -21.75,
+ "pos_z": 67.3125,
+ "stations": "Perry Dock"
+ },
+ {
+ "name": "Pemotenil",
+ "pos_x": 76.28125,
+ "pos_y": -122.40625,
+ "pos_z": 67.3125,
+ "stations": "Saha Installation,Osterbrock Base"
+ },
+ {
+ "name": "Penab",
+ "pos_x": -156.65625,
+ "pos_y": 0.875,
+ "pos_z": 60.125,
+ "stations": "Kizim Terminal,Reynolds Arsenal"
+ },
+ {
+ "name": "Penadak",
+ "pos_x": -69.375,
+ "pos_y": -1.90625,
+ "pos_z": 160.21875,
+ "stations": "Russell Station"
+ },
+ {
+ "name": "Penai",
+ "pos_x": -8.34375,
+ "pos_y": -185.25,
+ "pos_z": 58.1875,
+ "stations": "Oshima Dock,Gutierrez City,Parsons Port,Hughes Holdings"
+ },
+ {
+ "name": "Penapasoak",
+ "pos_x": 151.375,
+ "pos_y": -174.03125,
+ "pos_z": 37.53125,
+ "stations": "Neugebauer Port,Mitropoulos Laboratory,Kushida Orbital,Mason Mines,Mouhot Base"
+ },
+ {
+ "name": "Pencil Sector EL-Y d5",
+ "pos_x": 816.3125,
+ "pos_y": 2,
+ "pos_z": -44.03125,
+ "stations": "New Growth"
+ },
+ {
+ "name": "Pendii",
+ "pos_x": 111.15625,
+ "pos_y": 119.90625,
+ "pos_z": -60.78125,
+ "stations": "Borisenko Mines,Grimwood Bastion,Hurston Vision"
+ },
+ {
+ "name": "Pendijin",
+ "pos_x": 104,
+ "pos_y": -42.9375,
+ "pos_z": 36.34375,
+ "stations": "Hirn Port,Isaev Terminal"
+ },
+ {
+ "name": "Pendilla",
+ "pos_x": 15.59375,
+ "pos_y": 133.75,
+ "pos_z": 25.53125,
+ "stations": "Tyurin Orbital,Crampton Laboratory,Mullane Barracks"
+ },
+ {
+ "name": "Penef",
+ "pos_x": 77.4375,
+ "pos_y": 69.46875,
+ "pos_z": -84.4375,
+ "stations": "Pascal Dock,Skolem Holdings,Banks Installation"
+ },
+ {
+ "name": "Peneng Wa",
+ "pos_x": -33.8125,
+ "pos_y": -70.75,
+ "pos_z": -31.59375,
+ "stations": "Utkin's Folly,Szameit Ring,Julian Gateway,Carson Bastion"
+ },
+ {
+ "name": "Peng",
+ "pos_x": 84.8125,
+ "pos_y": -22.09375,
+ "pos_z": 87.53125,
+ "stations": "Stephens Bastion,Runco Hub,Lockhart Port,Lenthall Terminal,Clerk Enterprise"
+ },
+ {
+ "name": "Peng Dung",
+ "pos_x": -46.375,
+ "pos_y": -11.84375,
+ "pos_z": 92,
+ "stations": "Melvin Settlement,Coke Stop,Mallory Relay"
+ },
+ {
+ "name": "Pennsylvania",
+ "pos_x": -9513.9375,
+ "pos_y": -911.34375,
+ "pos_z": 19809.90625,
+ "stations": "The Pit"
+ },
+ {
+ "name": "Pens",
+ "pos_x": 162.8125,
+ "pos_y": 73.625,
+ "pos_z": 3.25,
+ "stations": "Manarov's Claim,Haberlandt Orbital,Jacobi Beacon,Silves Settlement"
+ },
+ {
+ "name": "Pentam",
+ "pos_x": 85.375,
+ "pos_y": -47.21875,
+ "pos_z": -129.59375,
+ "stations": "Doyle Vision,Busch Holdings,Ponce de Leon Colony"
+ },
+ {
+ "name": "Pentin",
+ "pos_x": -60.5625,
+ "pos_y": 53.375,
+ "pos_z": -162.75,
+ "stations": "Grego Reach,Hogan Mines"
+ },
+ {
+ "name": "Pents",
+ "pos_x": -154.5625,
+ "pos_y": -15.46875,
+ "pos_z": -63.6875,
+ "stations": "Bartoe Enterprise,Mendeleev City,Bolotov Installation,Kingsmill Settlement,Yaping Gateway"
+ },
+ {
+ "name": "Pentu",
+ "pos_x": 54.375,
+ "pos_y": 110.53125,
+ "pos_z": 48.5,
+ "stations": "Landsteiner Orbital"
+ },
+ {
+ "name": "Pentun",
+ "pos_x": 57.5625,
+ "pos_y": -5.1875,
+ "pos_z": -17.03125,
+ "stations": "Vinogradov Base,Bridges City,Baudry Prospect"
+ },
+ {
+ "name": "Penty",
+ "pos_x": -92,
+ "pos_y": -61.03125,
+ "pos_z": 55.15625,
+ "stations": "Boas Platform,Williams Camp"
+ },
+ {
+ "name": "Pepper",
+ "pos_x": -11.65625,
+ "pos_y": 29.875,
+ "pos_z": -57.71875,
+ "stations": "Pepper,Ehrlich Terminal"
+ },
+ {
+ "name": "Peque",
+ "pos_x": 124.875,
+ "pos_y": -179.59375,
+ "pos_z": 95.3125,
+ "stations": "Lalande Stop"
+ },
+ {
+ "name": "Pequen",
+ "pos_x": 58.75,
+ "pos_y": -163,
+ "pos_z": -32.71875,
+ "stations": "Sy Gateway,Geller Station,Fozard Enterprise,Brunner Gateway"
+ },
+ {
+ "name": "Pequenenses",
+ "pos_x": 147.4375,
+ "pos_y": -70.65625,
+ "pos_z": 83.78125,
+ "stations": "Hague Mines,Jackson Landing,Beshore Terminal,Anastase Perrotin Landing,Quinn Terminal"
+ },
+ {
+ "name": "Pera",
+ "pos_x": 30.59375,
+ "pos_y": -84.15625,
+ "pos_z": 63.03125,
+ "stations": "Hayashi City"
+ },
+ {
+ "name": "Peradjariu",
+ "pos_x": -99.125,
+ "pos_y": 23.28125,
+ "pos_z": 56.90625,
+ "stations": "Vries Port,Morris Dock,Beltrami Point"
+ },
+ {
+ "name": "Peraesii",
+ "pos_x": 69.5625,
+ "pos_y": -180.8125,
+ "pos_z": 36.59375,
+ "stations": "Giger Hub,Deslandres Orbital,Hickam Hub,Charlier Terminal,Danjon Port,Drummond Penal colony,Iben Dock,Trujillo Orbital,Elson Orbital,Espenak Port,Wilson Forum,Lemonnier Works,Skjellerup Landing,Minkowski Depot,Schmitz Base,Moran Prospect"
+ },
+ {
+ "name": "Peragi",
+ "pos_x": 37.75,
+ "pos_y": -129.21875,
+ "pos_z": 104.6875,
+ "stations": "Wafa Vision,Robert Dock,Babcock Hub,Frost Base,Barbaro Keep"
+ },
+ {
+ "name": "Peramongan",
+ "pos_x": -164.625,
+ "pos_y": -27.78125,
+ "pos_z": 45.375,
+ "stations": "Robinson Ring"
+ },
+ {
+ "name": "Perauni",
+ "pos_x": 150.03125,
+ "pos_y": 7.8125,
+ "pos_z": 59.625,
+ "stations": "Brooks Horizons,Gentle Platform,Griffith Installation"
+ },
+ {
+ "name": "Perce",
+ "pos_x": 146.40625,
+ "pos_y": -18.125,
+ "pos_z": 36.34375,
+ "stations": "Vlamingh Holdings,Mikulin Station,i Sola Landing,Gustav Sporer Colony"
+ },
+ {
+ "name": "Perci Bud",
+ "pos_x": -67.8125,
+ "pos_y": -41.8125,
+ "pos_z": -120.375,
+ "stations": "Onizuka Survey"
+ },
+ {
+ "name": "Percunceni",
+ "pos_x": 57.59375,
+ "pos_y": -93.84375,
+ "pos_z": 68.125,
+ "stations": "Reid Vision,Hulse Vision,Frobenius Installation,Lyne Laboratory"
+ },
+ {
+ "name": "Peredd",
+ "pos_x": 135.71875,
+ "pos_y": -39.53125,
+ "pos_z": 84.03125,
+ "stations": "Naubakht Base"
+ },
+ {
+ "name": "Peregrina",
+ "pos_x": -24.9375,
+ "pos_y": -80.59375,
+ "pos_z": -184.34375,
+ "stations": "Talos 1,Stabenow Reformatory,Talos 2"
+ },
+ {
+ "name": "Perendi",
+ "pos_x": -52.5625,
+ "pos_y": 33.09375,
+ "pos_z": -11.875,
+ "stations": "Liwei City,Singer Settlement"
+ },
+ {
+ "name": "Pereng Asli",
+ "pos_x": 172.8125,
+ "pos_y": -172.34375,
+ "pos_z": -63.65625,
+ "stations": "O'Neill Platform,Seyfert Dock,Grassmann Barracks"
+ },
+ {
+ "name": "Pergamon",
+ "pos_x": -9520.9375,
+ "pos_y": -896.65625,
+ "pos_z": 19808.78125,
+ "stations": "Malcolm Oasis"
+ },
+ {
+ "name": "Perkana",
+ "pos_x": -19.21875,
+ "pos_y": 42.5,
+ "pos_z": -75.6875,
+ "stations": "Dann Vision,Moon Station,Resnick Arsenal"
+ },
+ {
+ "name": "Perkele",
+ "pos_x": 58.875,
+ "pos_y": -23.5,
+ "pos_z": 4.40625,
+ "stations": "Checkpoint Brindtopia,Brenna's Legacy,Payne Gateway"
+ },
+ {
+ "name": "Perket",
+ "pos_x": 95.40625,
+ "pos_y": -43.78125,
+ "pos_z": -75.84375,
+ "stations": "Melvill Plant,Leoniceno Survey"
+ },
+ {
+ "name": "Perktomen",
+ "pos_x": 135.125,
+ "pos_y": 45.5625,
+ "pos_z": 0.3125,
+ "stations": "Rominger Station,Bhabha Penal colony,Wilson Enterprise"
+ },
+ {
+ "name": "Perkunas",
+ "pos_x": 2,
+ "pos_y": 50.09375,
+ "pos_z": 38.4375,
+ "stations": "Murdoch City,Hubble Gateway,van Vogt Base,Auer Horizons"
+ },
+ {
+ "name": "Perovii",
+ "pos_x": -52.78125,
+ "pos_y": 41.125,
+ "pos_z": 148.15625,
+ "stations": "Barnwell Prospect,Corte-Real Depot,Mohun Relay"
+ },
+ {
+ "name": "Perovoi",
+ "pos_x": -78.96875,
+ "pos_y": -67.21875,
+ "pos_z": 56.65625,
+ "stations": "Kerwin Holdings,Macquorn Rankine Terminal"
+ },
+ {
+ "name": "Persephone",
+ "pos_x": 31.59375,
+ "pos_y": -104.53125,
+ "pos_z": 8.0625,
+ "stations": "Lombardelli's Legacy"
+ },
+ {
+ "name": "Perun",
+ "pos_x": 15.9375,
+ "pos_y": 48.375,
+ "pos_z": 37.3125,
+ "stations": "Shukor Dock,Haipeng Gateway,Vittori Keep"
+ },
+ {
+ "name": "Pethes",
+ "pos_x": -20.65625,
+ "pos_y": -32.46875,
+ "pos_z": -11.90625,
+ "stations": "Yuzhe Port,Salam Dock"
+ },
+ {
+ "name": "Petra",
+ "pos_x": 14.9375,
+ "pos_y": -60.34375,
+ "pos_z": 66.96875,
+ "stations": "Salpeter Prospect,Bond Station,Aaronson Installation,Maybury Refinery,Oshima Hangar"
+ },
+ {
+ "name": "Pfembali",
+ "pos_x": 85.125,
+ "pos_y": -19.03125,
+ "pos_z": -70.125,
+ "stations": "Crown Enterprise,Dupuy de Lome Port,Lawhead Survey,Nachtigal Dock,Burstein's Inheritance"
+ },
+ {
+ "name": "Phanes",
+ "pos_x": 10.5,
+ "pos_y": 25.28125,
+ "pos_z": -47.34375,
+ "stations": "Mawson Oasis,Leiber Installation,Quimper Dock,Mendeleev Prospect,Heinlein Depot"
+ },
+ {
+ "name": "Phekda",
+ "pos_x": -25.0625,
+ "pos_y": 73.3125,
+ "pos_z": -30.28125,
+ "stations": "Wener Orbiter,Leckie Town,Fort Gonzalez,Lee Relay,Ousey City"
+ },
+ {
+ "name": "Phi Eridani",
+ "pos_x": 74.71875,
+ "pos_y": -134.1875,
+ "pos_z": 6.03125,
+ "stations": "Hill Survey,Arago Lab"
+ },
+ {
+ "name": "Phi Gruis",
+ "pos_x": 6.71875,
+ "pos_y": -106.34375,
+ "pos_z": 44.59375,
+ "stations": "Burbidge's Inheritance,Hirayama Depot"
+ },
+ {
+ "name": "Phi-1 Pavonis",
+ "pos_x": 29.40625,
+ "pos_y": -54.09375,
+ "pos_z": 66.53125,
+ "stations": "Offutt Stop,Anders Beacon"
+ },
+ {
+ "name": "Phiagre",
+ "pos_x": 44.28125,
+ "pos_y": -82.96875,
+ "pos_z": 52.5,
+ "stations": "Greeboski's Outpost,Hughes Port,Shinn Landing,Sopheos,The Victoria Chappell Foundation,Moskowitz Town"
+ },
+ {
+ "name": "Phiince",
+ "pos_x": 18.28125,
+ "pos_y": -29.03125,
+ "pos_z": -21.125,
+ "stations": "Chopper's Orbital"
+ },
+ {
+ "name": "Phoenix",
+ "pos_x": -9544.21875,
+ "pos_y": -906.84375,
+ "pos_z": 19830.28125,
+ "stations": "The Nest"
+ },
+ {
+ "name": "Phorcys",
+ "pos_x": 46.4375,
+ "pos_y": -38.09375,
+ "pos_z": -55.28125,
+ "stations": "Davidson Reformatory,Durrance Refinery,Schmitt Plant"
+ },
+ {
+ "name": "Phra Atah",
+ "pos_x": -3.15625,
+ "pos_y": -141.875,
+ "pos_z": 59.71875,
+ "stations": "Langley Terminal,Escobar Penal colony"
+ },
+ {
+ "name": "Phra Athi",
+ "pos_x": -110.875,
+ "pos_y": 0.5,
+ "pos_z": 6.71875,
+ "stations": "Oswald Base"
+ },
+ {
+ "name": "Phra Athit",
+ "pos_x": 4.46875,
+ "pos_y": -65.0625,
+ "pos_z": -72.28125,
+ "stations": "Jeschke Colony,Oramus Beacon"
+ },
+ {
+ "name": "Phra Gongo",
+ "pos_x": 85.9375,
+ "pos_y": -13.28125,
+ "pos_z": 60.75,
+ "stations": "Hartsfield Prospect,Pailes Depot"
+ },
+ {
+ "name": "Phra Gu",
+ "pos_x": -10.75,
+ "pos_y": 144.75,
+ "pos_z": -40.65625,
+ "stations": "Zebrowski Outpost,Hippalus Landing"
+ },
+ {
+ "name": "Phra Imish",
+ "pos_x": 96.15625,
+ "pos_y": 103.21875,
+ "pos_z": 49.46875,
+ "stations": "Nikolayev Works,Carr Stop,Peral Vision"
+ },
+ {
+ "name": "Phra Indji",
+ "pos_x": -17.0625,
+ "pos_y": -200.96875,
+ "pos_z": 42.09375,
+ "stations": "Wallis Platform"
+ },
+ {
+ "name": "Phra Lak",
+ "pos_x": 28.5,
+ "pos_y": 32.09375,
+ "pos_z": 5.3125,
+ "stations": "Janszoon Dock,Schiltberger Station"
+ },
+ {
+ "name": "Phra Lule",
+ "pos_x": 58.5,
+ "pos_y": -79.125,
+ "pos_z": -32.03125,
+ "stations": "Homer Plant,Rittenhouse Landing,Minkowski's Inheritance"
+ },
+ {
+ "name": "Phra Mool",
+ "pos_x": 25.0625,
+ "pos_y": -19.96875,
+ "pos_z": 58.875,
+ "stations": "Darlton Orbital"
+ },
+ {
+ "name": "Phra Narai",
+ "pos_x": 113.6875,
+ "pos_y": -69.1875,
+ "pos_z": -64.0625,
+ "stations": "Vuia Terminal,Maury Prospect,Brom Prospect"
+ },
+ {
+ "name": "Phra Ram",
+ "pos_x": -52.03125,
+ "pos_y": -29.71875,
+ "pos_z": 149.59375,
+ "stations": "Lockhart Dock,Delbruck Colony,Archimedes Dock"
+ },
+ {
+ "name": "Phra Saman",
+ "pos_x": 8.59375,
+ "pos_y": -78.3125,
+ "pos_z": -24.25,
+ "stations": "Longomontanus Dock,Silverberg Base"
+ },
+ {
+ "name": "Phra Sheng",
+ "pos_x": -69.8125,
+ "pos_y": 22.90625,
+ "pos_z": -119.46875,
+ "stations": "Verne Refinery,Kingsbury Hangar"
+ },
+ {
+ "name": "Phra Shenty",
+ "pos_x": 110.4375,
+ "pos_y": 101.28125,
+ "pos_z": 34.59375,
+ "stations": "Al-Battani Dock"
+ },
+ {
+ "name": "Phra Yai",
+ "pos_x": -66.375,
+ "pos_y": 74.15625,
+ "pos_z": 89.53125,
+ "stations": "Bao Dock,Wells Reach"
+ },
+ {
+ "name": "Phra Zhan",
+ "pos_x": 78.8125,
+ "pos_y": 56.0625,
+ "pos_z": 7.21875,
+ "stations": "Karl Diesel Ring,Lovell Dock"
+ },
+ {
+ "name": "Phra Zhons",
+ "pos_x": 87.90625,
+ "pos_y": -48.34375,
+ "pos_z": 9.625,
+ "stations": "Mitchell City,Zhigang Port,Dutton City,Glidden Penal colony"
+ },
+ {
+ "name": "Phracan",
+ "pos_x": 132.03125,
+ "pos_y": -36.03125,
+ "pos_z": -49.25,
+ "stations": "Foerster Stop"
+ },
+ {
+ "name": "Phracani",
+ "pos_x": 112.53125,
+ "pos_y": -116.03125,
+ "pos_z": -19.78125,
+ "stations": "Maunder Vision,Marriott Terminal,Lippisch Ring"
+ },
+ {
+ "name": "Phraces",
+ "pos_x": -101.875,
+ "pos_y": -102.875,
+ "pos_z": 15.84375,
+ "stations": "Potter Enterprise,Baxter Penal colony,Mullane Ring,Grechko Beacon"
+ },
+ {
+ "name": "Phrasika",
+ "pos_x": -26.84375,
+ "pos_y": -100.46875,
+ "pos_z": 57.84375,
+ "stations": "Anthony Prospect,Bohme's Folly,Windt Orbital,Hovgaard Prospect"
+ },
+ {
+ "name": "Phrasinigus",
+ "pos_x": 79.625,
+ "pos_y": -33.15625,
+ "pos_z": 147.25,
+ "stations": "Davies Prospect,Britnev Terminal,Wilhelm von Struve Holdings"
+ },
+ {
+ "name": "Phrygia",
+ "pos_x": 15.21875,
+ "pos_y": -31.25,
+ "pos_z": 87.28125,
+ "stations": "den Berg Hub,Nagata Bastion"
+ },
+ {
+ "name": "Phrygiang",
+ "pos_x": 66.9375,
+ "pos_y": -66.625,
+ "pos_z": -101.5625,
+ "stations": "Revin Hub,Chiao Enterprise"
+ },
+ {
+ "name": "Pi Dhod",
+ "pos_x": 99,
+ "pos_y": -76.75,
+ "pos_z": 85.8125,
+ "stations": "Seares Gateway,Sewell Vision,Furuta's Inheritance,Bartolomeu de Gusmao Dock"
+ },
+ {
+ "name": "Pi Dimo",
+ "pos_x": -96.28125,
+ "pos_y": 63.28125,
+ "pos_z": 116.53125,
+ "stations": "Soddy Port,Hardwick Enterprise"
+ },
+ {
+ "name": "Pi Dimshi",
+ "pos_x": -140,
+ "pos_y": 4.1875,
+ "pos_z": -89.03125,
+ "stations": "Crown Orbital,Janszoon's Inheritance,Dirichlet Penal colony"
+ },
+ {
+ "name": "Pi Disci",
+ "pos_x": 37.46875,
+ "pos_y": -3.78125,
+ "pos_z": -102.78125,
+ "stations": "Lockhart Relay"
+ },
+ {
+ "name": "Pi Ma Wen",
+ "pos_x": 164.65625,
+ "pos_y": -61.53125,
+ "pos_z": -0.125,
+ "stations": "Naburimannu Port"
+ },
+ {
+ "name": "Pi Mensae",
+ "pos_x": 47.78125,
+ "pos_y": -29.84375,
+ "pos_z": 19.8125,
+ "stations": "Trueman's Rest,Gelfand Orbital"
+ },
+ {
+ "name": "Pi Pavonis",
+ "pos_x": 59.71875,
+ "pos_y": -44.65625,
+ "pos_z": 106.46875,
+ "stations": "Lagrange Hub,Meaney Enterprise,Ferguson Exchange,Rawn Ring"
+ },
+ {
+ "name": "Pi Piscis Austrini",
+ "pos_x": -5.625,
+ "pos_y": -87.90625,
+ "pos_z": 38.09375,
+ "stations": "Laming Station,Farghani Hub,al-Kashi Terminal,Taylor Jr. Station,Hussenot Mine,Hipparchus Port,Louis de Lacaille Terminal,Springer Base,Ulloa Dock,Foster's Folly,Pond Beacon"
+ },
+ {
+ "name": "Pi Sculptoris",
+ "pos_x": 40.0625,
+ "pos_y": -209.78125,
+ "pos_z": -23.75,
+ "stations": "Kelly Port,Theodor Winnecke Dock,Finlay-Freundlich Outpost,Roe Base,Sverdrup Arena"
+ },
+ {
+ "name": "Pi-fang",
+ "pos_x": -34.65625,
+ "pos_y": 22.84375,
+ "pos_z": -4.59375,
+ "stations": "Brooks Estate,Cartier's Port,Napier Settlement,Piaget Prospect,Quinn Dock"
+ },
+ {
+ "name": "Piapok",
+ "pos_x": 0.46875,
+ "pos_y": -1.90625,
+ "pos_z": -160.625,
+ "stations": "Henslow's Inheritance"
+ },
+ {
+ "name": "Piaponnus",
+ "pos_x": -107.71875,
+ "pos_y": 37.71875,
+ "pos_z": 47.6875,
+ "stations": "Reiter Terminal"
+ },
+ {
+ "name": "Piapoyo",
+ "pos_x": 47.34375,
+ "pos_y": -211.9375,
+ "pos_z": 113.40625,
+ "stations": "Lopez-Garcia Camp"
+ },
+ {
+ "name": "Piar",
+ "pos_x": 49.25,
+ "pos_y": -128.6875,
+ "pos_z": 82.03125,
+ "stations": "Binney Station,Bolden Penal colony,Albategnius Station,Anderson Enterprise"
+ },
+ {
+ "name": "Piara",
+ "pos_x": 155.96875,
+ "pos_y": -120.28125,
+ "pos_z": 58.5,
+ "stations": "Fischer Depot"
+ },
+ {
+ "name": "Pibe",
+ "pos_x": 106.5,
+ "pos_y": -40.96875,
+ "pos_z": 98.09375,
+ "stations": "Whittle Vision,Struzan Vision,Oshima Station"
+ },
+ {
+ "name": "Pibelmen",
+ "pos_x": -93.6875,
+ "pos_y": -143.46875,
+ "pos_z": -27.125,
+ "stations": "Ito Vision,Belyanin Bastion"
+ },
+ {
+ "name": "Pibes",
+ "pos_x": 115.96875,
+ "pos_y": -74.8125,
+ "pos_z": -48.90625,
+ "stations": "McManus Outpost,Proctor Survey"
+ },
+ {
+ "name": "Pic Tok",
+ "pos_x": -81.46875,
+ "pos_y": -22.875,
+ "pos_z": -160.84375,
+ "stations": "Lobachevsky Outpost"
+ },
+ {
+ "name": "Pican",
+ "pos_x": 1.75,
+ "pos_y": -127.875,
+ "pos_z": -91.375,
+ "stations": "Robinson Prospect,Ford Dock,Starzl Relay"
+ },
+ {
+ "name": "Picaure",
+ "pos_x": 116.375,
+ "pos_y": -44.25,
+ "pos_z": -39.03125,
+ "stations": "Hand's Pride"
+ },
+ {
+ "name": "Picaurukan",
+ "pos_x": 21.90625,
+ "pos_y": -74.03125,
+ "pos_z": -18.6875,
+ "stations": "Cunningham City,Aristotle Terminal,Bean Gateway,Gamow Hub,Laliberte Keep,Morukov Gateway,Clerk Station,Bamford Prospect,Thompson Enterprise"
+ },
+ {
+ "name": "Picenile",
+ "pos_x": 135.40625,
+ "pos_y": 10.71875,
+ "pos_z": -93,
+ "stations": "Crippen Dock"
+ },
+ {
+ "name": "Picenth",
+ "pos_x": -60.90625,
+ "pos_y": -7.8125,
+ "pos_z": 139.46875,
+ "stations": "Landis Landing,Pullman Mines,Meitner's Folly"
+ },
+ {
+ "name": "Pices",
+ "pos_x": -70.40625,
+ "pos_y": -22.375,
+ "pos_z": 63.09375,
+ "stations": "Smith Bastion,Knipling Port,Holden Base,Pontes Port,Rothman Works,Merchiston Laboratory"
+ },
+ {
+ "name": "Pichanto",
+ "pos_x": 92.25,
+ "pos_y": 12.84375,
+ "pos_z": -26.5,
+ "stations": "Thorne Landing,Onnes Stop"
+ },
+ {
+ "name": "Pichch",
+ "pos_x": -47.59375,
+ "pos_y": -128.6875,
+ "pos_z": -36.03125,
+ "stations": "Tiptree Port,Besonders City,Baffin Platform"
+ },
+ {
+ "name": "Pichi Wang",
+ "pos_x": -24.59375,
+ "pos_y": -101.09375,
+ "pos_z": 116.46875,
+ "stations": "Slonczewski Dock,Sohl Dock,Block Platform,Haarsma's Progress"
+ },
+ {
+ "name": "Pichua",
+ "pos_x": 163.8125,
+ "pos_y": 0.59375,
+ "pos_z": 62.03125,
+ "stations": "Key Camp,Csoma Relay,Nemere's Claim"
+ },
+ {
+ "name": "Picoria",
+ "pos_x": 92.59375,
+ "pos_y": -84.71875,
+ "pos_z": -25.96875,
+ "stations": "Schomburg Terminal,Wolszczan Terminal,Kohoutek Ring,Binder Landing,Jones Keep,Schumacher's Progress"
+ },
+ {
+ "name": "Pictavit",
+ "pos_x": -133.96875,
+ "pos_y": 53.15625,
+ "pos_z": -10.65625,
+ "stations": "Ansari Colony"
+ },
+ {
+ "name": "Pictavul",
+ "pos_x": 71.78125,
+ "pos_y": -171.3125,
+ "pos_z": 102.84375,
+ "stations": "Airy Ring,Merrill Terminal"
+ },
+ {
+ "name": "Piculta",
+ "pos_x": -7.53125,
+ "pos_y": -69.28125,
+ "pos_z": 49,
+ "stations": "Olsen Dock"
+ },
+ {
+ "name": "Picunche",
+ "pos_x": -2.6875,
+ "pos_y": 25.40625,
+ "pos_z": 113.15625,
+ "stations": "Bartoe City,Bell Terminal,Amundsen Base,Schilling Survey,Noether Arena,Shatalov Point"
+ },
+ {
+ "name": "Picuni",
+ "pos_x": -43.53125,
+ "pos_y": -131.34375,
+ "pos_z": 3.21875,
+ "stations": "Arrhenius Gateway,Nilson Horizons,Artin Landing"
+ },
+ {
+ "name": "Picus",
+ "pos_x": 100.59375,
+ "pos_y": 12.84375,
+ "pos_z": -32.03125,
+ "stations": "Flade Works,Lagrange Platform,Baird Plant"
+ },
+ {
+ "name": "Pie",
+ "pos_x": -35.375,
+ "pos_y": 77.65625,
+ "pos_z": -9.125,
+ "stations": "Sarmiento de Gamboa Settlement,Bester Hangar"
+ },
+ {
+ "name": "Piegfrielta",
+ "pos_x": -69.46875,
+ "pos_y": -68.125,
+ "pos_z": 111.46875,
+ "stations": "Abnett Platform,Bartlett Colony"
+ },
+ {
+ "name": "Piegua",
+ "pos_x": -119.53125,
+ "pos_y": 0.53125,
+ "pos_z": 139.03125,
+ "stations": "Grandin Hub,Newman Terminal"
+ },
+ {
+ "name": "Pien Ek",
+ "pos_x": -15.4375,
+ "pos_y": -16.46875,
+ "pos_z": -149.28125,
+ "stations": "Svavarsson Survey"
+ },
+ {
+ "name": "Pien Nik",
+ "pos_x": -32.59375,
+ "pos_y": -183.65625,
+ "pos_z": 47.6875,
+ "stations": "Coye Landing,Shajn Beacon,Levinson Bastion,Milnor Prospect"
+ },
+ {
+ "name": "Pien Tsach",
+ "pos_x": -58.5,
+ "pos_y": -33.53125,
+ "pos_z": -12.15625,
+ "stations": "Atwater Station"
+ },
+ {
+ "name": "Pienapa",
+ "pos_x": 77.0625,
+ "pos_y": -258.375,
+ "pos_z": 17.21875,
+ "stations": "Busemann Base,Gann Hub"
+ },
+ {
+ "name": "Pienate",
+ "pos_x": -17.3125,
+ "pos_y": 8.34375,
+ "pos_z": 58.75,
+ "stations": "Walz Ring,Sacco Hub,Libby City"
+ },
+ {
+ "name": "Pijaberet",
+ "pos_x": -117.4375,
+ "pos_y": 22.53125,
+ "pos_z": 97.75,
+ "stations": "Sarich Station"
+ },
+ {
+ "name": "Pijan",
+ "pos_x": 8.8125,
+ "pos_y": 58.65625,
+ "pos_z": 114.71875,
+ "stations": "Rice Station,Amis Orbital,Adams Installation"
+ },
+ {
+ "name": "Pijao",
+ "pos_x": 21.96875,
+ "pos_y": 66.84375,
+ "pos_z": 150.34375,
+ "stations": "Andersson Orbital,Kirtley Horizons,Herreshoff Point,Farouk Landing"
+ },
+ {
+ "name": "Pikudiyung",
+ "pos_x": -63.59375,
+ "pos_y": 65.96875,
+ "pos_z": -99.96875,
+ "stations": "Blish City,Rothman Installation,Tucker Holdings,Davidson Barracks"
+ },
+ {
+ "name": "Pikum",
+ "pos_x": -39,
+ "pos_y": -63.125,
+ "pos_z": -75.03125,
+ "stations": "Prunariu Gateway,Rushworth Station,Feoktistov Platform,Martinez Reach,Watts Hub"
+ },
+ {
+ "name": "Pilakulaman",
+ "pos_x": 48.375,
+ "pos_y": -76.625,
+ "pos_z": 168.34375,
+ "stations": "Burgess Camp"
+ },
+ {
+ "name": "Pilatapa",
+ "pos_x": -35.9375,
+ "pos_y": 72.96875,
+ "pos_z": 144.21875,
+ "stations": "Meyrink Station,Hugh Point"
+ },
+ {
+ "name": "Pilati",
+ "pos_x": 29,
+ "pos_y": -104.4375,
+ "pos_z": -54.6875,
+ "stations": "Wild Station,Kurtz Vision"
+ },
+ {
+ "name": "Pilatock",
+ "pos_x": 52.5,
+ "pos_y": -24.59375,
+ "pos_z": -61.53125,
+ "stations": "Khrunov Hub,Jones' Pride,Hoften Terminal"
+ },
+ {
+ "name": "Pilatucates",
+ "pos_x": 105.375,
+ "pos_y": -75,
+ "pos_z": -78,
+ "stations": "Whitson Colony,Roosa Laboratory"
+ },
+ {
+ "name": "Pilintana",
+ "pos_x": 100.5,
+ "pos_y": 0.65625,
+ "pos_z": 28,
+ "stations": "Noli Landing,Gentle Port,Tanner Station,Savorgnan de Brazza Holdings,Norton's Folly"
+ },
+ {
+ "name": "Piliqui",
+ "pos_x": -39.625,
+ "pos_y": -109.375,
+ "pos_z": 31.5,
+ "stations": "Dixon Station,Kirtley Penal colony"
+ },
+ {
+ "name": "Piliwa",
+ "pos_x": 56.71875,
+ "pos_y": 98.46875,
+ "pos_z": -79.0625,
+ "stations": "Benyovszky's Claim"
+ },
+ {
+ "name": "Pilnga",
+ "pos_x": 70.625,
+ "pos_y": -155.78125,
+ "pos_z": 71.21875,
+ "stations": "Bradfield Hub,Ortiz Moreno Base"
+ },
+ {
+ "name": "Pilngalu",
+ "pos_x": 48.1875,
+ "pos_y": 32.53125,
+ "pos_z": 56.09375,
+ "stations": "Boyle Station"
+ },
+ {
+ "name": "Pima",
+ "pos_x": -126.21875,
+ "pos_y": 86.5625,
+ "pos_z": 26.1875,
+ "stations": "Reynolds Installation,Bernoulli Settlement,Martinez Terminal,Brunner Point"
+ },
+ {
+ "name": "Pimari",
+ "pos_x": 62.34375,
+ "pos_y": -130.84375,
+ "pos_z": 185.96875,
+ "stations": "Penrose Beacon"
+ },
+ {
+ "name": "Pimarisi",
+ "pos_x": 59.5,
+ "pos_y": 33.84375,
+ "pos_z": -79.4375,
+ "stations": "Culpeper Platform,Appel Base"
+ },
+ {
+ "name": "Pindi",
+ "pos_x": -42.375,
+ "pos_y": 143.3125,
+ "pos_z": -98.125,
+ "stations": "Bellamy Reach,Lunan Base"
+ },
+ {
+ "name": "Pindians",
+ "pos_x": 73.96875,
+ "pos_y": -194.40625,
+ "pos_z": 55.78125,
+ "stations": "Dean Vision,Borrelly Orbital,Velho's Progress"
+ },
+ {
+ "name": "Pindilla",
+ "pos_x": 30.8125,
+ "pos_y": -174.84375,
+ "pos_z": 100.6875,
+ "stations": "Flammarion Terminal,Waldrop Arena"
+ },
+ {
+ "name": "Pindjeri",
+ "pos_x": 167.40625,
+ "pos_y": -163.53125,
+ "pos_z": -6.03125,
+ "stations": "Whittle Mine,Franklin Escape"
+ },
+ {
+ "name": "Pinduma",
+ "pos_x": -93.75,
+ "pos_y": -43.28125,
+ "pos_z": 27.5625,
+ "stations": "Vernadsky Depot,Parise Landing"
+ },
+ {
+ "name": "Pingar",
+ "pos_x": 55.4375,
+ "pos_y": -102.71875,
+ "pos_z": 118.75,
+ "stations": "d'Allonville Platform,Urata Survey,Barmin Vision"
+ },
+ {
+ "name": "Pingga",
+ "pos_x": 42.59375,
+ "pos_y": 22.4375,
+ "pos_z": 65.6875,
+ "stations": "Banks Dock"
+ },
+ {
+ "name": "Pinggul",
+ "pos_x": 145.34375,
+ "pos_y": -215.0625,
+ "pos_z": 50.25,
+ "stations": "Smirnova Platform,Francis Arena"
+ },
+ {
+ "name": "Pingurasin",
+ "pos_x": -78,
+ "pos_y": 118.65625,
+ "pos_z": -83.59375,
+ "stations": "Robinson Camp,Godwin Retreat,Davidson Beacon"
+ },
+ {
+ "name": "Pini",
+ "pos_x": 121.8125,
+ "pos_y": -55.90625,
+ "pos_z": 37.125,
+ "stations": "Florine Ring,Czerneda Installation,Arzachel Station,Vaisala Orbital,Shoujing Orbital,Plancius Orbital,Glushko Orbital,Baille Station,Fraknoi Gateway,Allen Vista,Crown's Pride,Boyer Vision,Kirby Port,Gonnessiat Landing,Barreiros Prospect"
+ },
+ {
+ "name": "Pinibo",
+ "pos_x": -44.40625,
+ "pos_y": -56.71875,
+ "pos_z": 144.65625,
+ "stations": "Martins Settlement,Hovgaard Horizons,Rothman Station,Maine Prospect"
+ },
+ {
+ "name": "Pinouphis",
+ "pos_x": -88.03125,
+ "pos_y": 13.96875,
+ "pos_z": 2.5625,
+ "stations": "Stephens Hub,Stableford Dock,Watts Dock"
+ },
+ {
+ "name": "Pintec",
+ "pos_x": 56.25,
+ "pos_y": -181.5625,
+ "pos_z": -86.90625,
+ "stations": "Paola Point"
+ },
+ {
+ "name": "Pintu",
+ "pos_x": 104.6875,
+ "pos_y": -161.375,
+ "pos_z": -20.96875,
+ "stations": "Dawes Station,Sharp Holdings,Penzias Orbital"
+ },
+ {
+ "name": "Piorimudjar",
+ "pos_x": 107.8125,
+ "pos_y": -76.40625,
+ "pos_z": 33.25,
+ "stations": "Bauman Terminal,Asami Market,Gotlieb Enterprise,Gunter Terminal,Sagan Terminal,Delaunay Vision,Brunner Horizons,Ludwig Struve Dock,Chacornac Orbital,Zhukovsky Palace"
+ },
+ {
+ "name": "Piorung",
+ "pos_x": -12.625,
+ "pos_y": 129.1875,
+ "pos_z": -36.28125,
+ "stations": "Miletus Penal colony,Diamond Platform"
+ },
+ {
+ "name": "Pipedu",
+ "pos_x": -126.5,
+ "pos_y": 47.8125,
+ "pos_z": -82.71875,
+ "stations": "Bao Outpost"
+ },
+ {
+ "name": "Pipera",
+ "pos_x": 76.03125,
+ "pos_y": 78.40625,
+ "pos_z": 76.5,
+ "stations": "Sagan Hub,King Vision,Tilley Holdings"
+ },
+ {
+ "name": "Piperi",
+ "pos_x": -136.78125,
+ "pos_y": -17.40625,
+ "pos_z": 108.875,
+ "stations": "Dayuan Beacon"
+ },
+ {
+ "name": "Piperish",
+ "pos_x": 52.46875,
+ "pos_y": -183.34375,
+ "pos_z": 20.28125,
+ "stations": "Moorcock Enterprise,Gloss Vision,Ziemianski Hub,Bombelli Horizons,Theodor Winnecke Horizons,Bauschinger Horizons,O'Leary Keep"
+ },
+ {
+ "name": "Pipet",
+ "pos_x": -158.53125,
+ "pos_y": -15.28125,
+ "pos_z": 17.78125,
+ "stations": "Velazquez Enterprise,Popov Orbital,Scobee Holdings"
+ },
+ {
+ "name": "Pipi",
+ "pos_x": -48.25,
+ "pos_y": -113.03125,
+ "pos_z": 77.8125,
+ "stations": "England Port,Carson Terminal,Libby Hub,de Sousa Horizons,Volk Enterprise,Zewail Dock"
+ },
+ {
+ "name": "Pipia",
+ "pos_x": -62.34375,
+ "pos_y": -134.21875,
+ "pos_z": -47.90625,
+ "stations": "Greenland Outpost"
+ },
+ {
+ "name": "Pipinoukhe",
+ "pos_x": 157.25,
+ "pos_y": -184.3125,
+ "pos_z": 55.4375,
+ "stations": "Fancher's Inheritance,Dominique Hub,Kuchner Dock"
+ },
+ {
+ "name": "Pipitei",
+ "pos_x": 135.15625,
+ "pos_y": -104.6875,
+ "pos_z": -42.15625,
+ "stations": "Lunney Port,Lintott Dock,Finney Barracks,Roe Terminal"
+ },
+ {
+ "name": "Pira",
+ "pos_x": 139.15625,
+ "pos_y": -140.34375,
+ "pos_z": 78.75,
+ "stations": "Kraft Depot,Kuo Port"
+ },
+ {
+ "name": "Piran",
+ "pos_x": -124.40625,
+ "pos_y": 39.5,
+ "pos_z": -13.96875,
+ "stations": "Kandel Settlement,Meikle Gateway,White Enterprise,Schottky Terminal,Metz's Inheritance,Khan Dock,Nicollier Terminal,Paez Vision"
+ },
+ {
+ "name": "Pirantae",
+ "pos_x": 142.46875,
+ "pos_y": -97.625,
+ "pos_z": 50.625,
+ "stations": "Dunlop Terminal,Parsons Dock,Brunner Dock,Khrenov's Inheritance"
+ },
+ {
+ "name": "Pirawilotri",
+ "pos_x": 17.84375,
+ "pos_y": -98.8125,
+ "pos_z": -89.28125,
+ "stations": "Jefferies Platform,Gurshtein Plant,Bertin Depot,Evangelisti Camp"
+ },
+ {
+ "name": "Pirawoth",
+ "pos_x": -19.375,
+ "pos_y": -0.03125,
+ "pos_z": -95.1875,
+ "stations": "Guerrero's Progress,Hennen Port,Wilson's Inheritance,Mohri Hub"
+ },
+ {
+ "name": "Pirawoyn",
+ "pos_x": -130.59375,
+ "pos_y": 32.5625,
+ "pos_z": -2.3125,
+ "stations": "Smith Refinery,Northrop Survey"
+ },
+ {
+ "name": "Pirigen",
+ "pos_x": 169.53125,
+ "pos_y": -138.09375,
+ "pos_z": 9.1875,
+ "stations": "Iben Dock,Barlowe Dock,Sagan's Folly"
+ },
+ {
+ "name": "Piriti",
+ "pos_x": -47.9375,
+ "pos_y": -1.1875,
+ "pos_z": -106.6875,
+ "stations": "Fadlan Enterprise,Gromov Dock,Rennie Hub,Junlong Installation"
+ },
+ {
+ "name": "Pirni",
+ "pos_x": 83.09375,
+ "pos_y": 104.25,
+ "pos_z": 89.3125,
+ "stations": "Knapp Point,Blackwell Settlement,Xing Survey"
+ },
+ {
+ "name": "Piruba",
+ "pos_x": 115.875,
+ "pos_y": -186.0625,
+ "pos_z": -23.125,
+ "stations": "Hind Mine"
+ },
+ {
+ "name": "Pisage",
+ "pos_x": 85.4375,
+ "pos_y": -236.6875,
+ "pos_z": -34.46875,
+ "stations": "Dugan Terminal,Stephenson Point,i Sola Relay"
+ },
+ {
+ "name": "Pisagi",
+ "pos_x": 75.59375,
+ "pos_y": -51.84375,
+ "pos_z": 80,
+ "stations": "Langley Mine"
+ },
+ {
+ "name": "Pisagii",
+ "pos_x": 83.625,
+ "pos_y": -60.09375,
+ "pos_z": 122.09375,
+ "stations": "Gorey Port,Rizvi Terminal"
+ },
+ {
+ "name": "Pisakas",
+ "pos_x": 162.40625,
+ "pos_y": -76.53125,
+ "pos_z": 47.71875,
+ "stations": "Hughes Camp,Chretien Oudemans Mines"
+ },
+ {
+ "name": "Pisaly",
+ "pos_x": 47.09375,
+ "pos_y": 24.03125,
+ "pos_z": -110.28125,
+ "stations": "Caryanda Dock,Bryusov Dock,Goldstein Terminal,Fast Port,Carey Settlement,Hedin Vision,MacLeod Depot,Kapp Hub,Szentmartony Enterprise,Noon Enterprise,Stackpole Prospect,Effinger Ring"
+ },
+ {
+ "name": "Pisamazin",
+ "pos_x": 84.9375,
+ "pos_y": -30.8125,
+ "pos_z": 96.375,
+ "stations": "Lomonosov Ring,Ghez City,Parkinson Terminal,Noether Vision"
+ },
+ {
+ "name": "Pisat",
+ "pos_x": 172.5625,
+ "pos_y": 41.4375,
+ "pos_z": 41.71875,
+ "stations": "Mackenzie Port"
+ },
+ {
+ "name": "Piscium Sector BQ-Y b0",
+ "pos_x": -68.8125,
+ "pos_y": -66.25,
+ "pos_z": -79.96875,
+ "stations": "Gaspar de Portola Works"
+ },
+ {
+ "name": "Piscium Sector GH-V b2-0",
+ "pos_x": -100.21875,
+ "pos_y": -62.71875,
+ "pos_z": -44.125,
+ "stations": "Gerrold Beacon"
+ },
+ {
+ "name": "Piscium Sector GR-W c1-20",
+ "pos_x": -75.9375,
+ "pos_y": -98.90625,
+ "pos_z": -13.375,
+ "stations": "Davidson Escape,Borel Mines,Hovell Landing"
+ },
+ {
+ "name": "Piscium Sector IC-V b2-6",
+ "pos_x": -96.28125,
+ "pos_y": -66.0625,
+ "pos_z": -34.03125,
+ "stations": "Russ Terminal,Farkas Beacon,Coulomb Base"
+ },
+ {
+ "name": "Pitaniang",
+ "pos_x": 96.625,
+ "pos_y": -30.125,
+ "pos_z": -54.34375,
+ "stations": "Armstrong Hub"
+ },
+ {
+ "name": "Pitjang",
+ "pos_x": 159.65625,
+ "pos_y": -22.59375,
+ "pos_z": -4.90625,
+ "stations": "Agassiz Outpost,Kidman Survey,Aguirre Base"
+ },
+ {
+ "name": "Pitjari",
+ "pos_x": 99.8125,
+ "pos_y": -92.40625,
+ "pos_z": -77.125,
+ "stations": "Malerba Landing,Weiss Hangar,Payson Holdings"
+ },
+ {
+ "name": "Pleiades Sector AB-W b2-4",
+ "pos_x": -137.5625,
+ "pos_y": -118.25,
+ "pos_z": -380.4375,
+ "stations": "Noctrach-Ihazevich Research Facility"
+ },
+ {
+ "name": "Pleiades Sector DL-Y d65",
+ "pos_x": -83.28125,
+ "pos_y": -151.40625,
+ "pos_z": -347.1875,
+ "stations": "Agricola's Ascent"
+ },
+ {
+ "name": "Pleiades Sector GW-W c1-13",
+ "pos_x": -77.375,
+ "pos_y": -156.3125,
+ "pos_z": -352.03125,
+ "stations": "Cornell's Garden"
+ },
+ {
+ "name": "Pleiades Sector HR-W d1-41",
+ "pos_x": -83.96875,
+ "pos_y": -146.15625,
+ "pos_z": -334.21875,
+ "stations": "Malthus Terminal,Rescue Ship - Malthus Terminal"
+ },
+ {
+ "name": "Pleiades Sector HR-W d1-57",
+ "pos_x": -86.40625,
+ "pos_y": -125.15625,
+ "pos_z": -301.84375,
+ "stations": "PRE Logistics Support Zeta,Cleaver Prospect,Rescue Ship - Cleaver Prospect"
+ },
+ {
+ "name": "Pleiades Sector HR-W d1-74",
+ "pos_x": -87.40625,
+ "pos_y": -150.90625,
+ "pos_z": -344.96875,
+ "stations": "Corrigan Terminal"
+ },
+ {
+ "name": "Pleiades Sector HR-W d1-79",
+ "pos_x": -80.625,
+ "pos_y": -146.65625,
+ "pos_z": -343.25,
+ "stations": "The Penitent"
+ },
+ {
+ "name": "Pleiades Sector IH-V c2-16",
+ "pos_x": -92,
+ "pos_y": -143.5,
+ "pos_z": -341.3125,
+ "stations": "PRE Laboratory,TII Research Facility"
+ },
+ {
+ "name": "Pleiades Sector IH-V c2-5",
+ "pos_x": -78.71875,
+ "pos_y": -138.03125,
+ "pos_z": -321.78125,
+ "stations": "PRE Logistics Support Gamma,Rix Depot"
+ },
+ {
+ "name": "Pleiades Sector IH-V c2-7",
+ "pos_x": -78.46875,
+ "pos_y": -144.9375,
+ "pos_z": -324.9375,
+ "stations": "Borrego's Vision,Rescue Ship - Borrego's Vision"
+ },
+ {
+ "name": "Pleiades Sector IR-W d1-55",
+ "pos_x": -63.59375,
+ "pos_y": -147.40625,
+ "pos_z": -319.09375,
+ "stations": "The Oracle,Donar's Oak,Rescue Ship - The Oracle"
+ },
+ {
+ "name": "Pleiades Sector JC-V d2-62",
+ "pos_x": -82.1875,
+ "pos_y": -100.9375,
+ "pos_z": -260.59375,
+ "stations": "PRE Logistics Support Epsilon,Asami Orbital,Rescue Ship - Asami Orbital"
+ },
+ {
+ "name": "Pleiades Sector KC-V c2-11",
+ "pos_x": -86.59375,
+ "pos_y": -147.03125,
+ "pos_z": -339.28125,
+ "stations": "Kipling Orbital,Rescue Ship - Kipling Orbital"
+ },
+ {
+ "name": "Pleiades Sector KC-V c2-4",
+ "pos_x": -88.8125,
+ "pos_y": -148.71875,
+ "pos_z": -321.1875,
+ "stations": "Oort Orbital"
+ },
+ {
+ "name": "Pleiades Sector OI-T c3-7",
+ "pos_x": -69.4375,
+ "pos_y": -180.4375,
+ "pos_z": -287.625,
+ "stations": "Orcus Crag"
+ },
+ {
+ "name": "Pleiades Sector PD-S b4-0",
+ "pos_x": -80.625,
+ "pos_y": -156.6875,
+ "pos_z": -337.4375,
+ "stations": "Ieyasu Dock"
+ },
+ {
+ "name": "Pleione",
+ "pos_x": -77,
+ "pos_y": -146.78125,
+ "pos_z": -344.125,
+ "stations": "Stargazer"
+ },
+ {
+ "name": "Plendovii",
+ "pos_x": -71.0625,
+ "pos_y": 10.125,
+ "pos_z": 37.34375,
+ "stations": "Huxley Dock,Steakley Dock,Carleson Dock"
+ },
+ {
+ "name": "Plentauri",
+ "pos_x": -0.125,
+ "pos_y": -132.5,
+ "pos_z": -26.90625,
+ "stations": "Hay Installation,Schade Beacon,Aoki Station,Narvaez's Inheritance,Goodman Installation"
+ },
+ {
+ "name": "Pleutarama",
+ "pos_x": 105.46875,
+ "pos_y": -121.125,
+ "pos_z": 45.15625,
+ "stations": "Ball Orbital,Karman Vision"
+ },
+ {
+ "name": "Pleutef",
+ "pos_x": 36.90625,
+ "pos_y": 64.34375,
+ "pos_z": 85.6875,
+ "stations": "Normand Mines,Sheckley's Inheritance"
+ },
+ {
+ "name": "Pliny",
+ "pos_x": 4.625,
+ "pos_y": 36,
+ "pos_z": -13.5,
+ "stations": "Laplace Port,Carpenter City,Meitner Dock,Kandel Enterprise,Vespucci Silo"
+ },
+ {
+ "name": "Plutarch",
+ "pos_x": 6.15625,
+ "pos_y": -20.53125,
+ "pos_z": 31.96875,
+ "stations": "Busch City,Vlamingh Market,Cramer Dock,Klink Ring,Irens Hub,King Holdings,Hoshide Survey,Dall Enterprise,Siodmak Terminal,Hodgkinson Orbital,Al-Khalili Plant,Kneale Station,King Arsenal,Walter Vision,Runco Beacon"
+ },
+ {
+ "name": "PLX 695",
+ "pos_x": -43.34375,
+ "pos_y": -4.71875,
+ "pos_z": -62.5,
+ "stations": "Dover,Bursch Dock"
+ },
+ {
+ "name": "PMD2009 48",
+ "pos_x": 594.90625,
+ "pos_y": -431.4375,
+ "pos_z": -1071.78125,
+ "stations": "Orion Nebula Tourist Centre"
+ },
+ {
+ "name": "Poe",
+ "pos_x": -9496.25,
+ "pos_y": -920.3125,
+ "pos_z": 19803.78125,
+ "stations": "Neon Sanctuary"
+ },
+ {
+ "name": "Poemaku",
+ "pos_x": 38.3125,
+ "pos_y": 10.21875,
+ "pos_z": 100.25,
+ "stations": "Sladek Station,Bendell Hub,Daley Hub,Walker Vision"
+ },
+ {
+ "name": "Poemavati",
+ "pos_x": 27.40625,
+ "pos_y": 32.875,
+ "pos_z": 156.84375,
+ "stations": "Fermi Terminal,Diesel Terminal,Erikson Enterprise"
+ },
+ {
+ "name": "Pohnpet",
+ "pos_x": 67.8125,
+ "pos_y": -43.40625,
+ "pos_z": -44.59375,
+ "stations": "Dahm Vision,Hogan Penal colony,Houtman Observatory"
+ },
+ {
+ "name": "Pokomovoi",
+ "pos_x": -124.9375,
+ "pos_y": 85.34375,
+ "pos_z": -12.34375,
+ "stations": "al-Din City,Land Station,Barnes Base,Arnarson Holdings"
+ },
+ {
+ "name": "Pokoto",
+ "pos_x": -144.28125,
+ "pos_y": -53.25,
+ "pos_z": -69.0625,
+ "stations": "Schouten Terminal,Wang Gateway"
+ },
+ {
+ "name": "Pola",
+ "pos_x": 134.9375,
+ "pos_y": 28.4375,
+ "pos_z": -64.5,
+ "stations": "Shepherd Landing,Drew Installation,Lucretius Plant"
+ },
+ {
+ "name": "Polabians",
+ "pos_x": 114.125,
+ "pos_y": -173.71875,
+ "pos_z": 25.6875,
+ "stations": "Bond Horizons,Hulse Landing,Harding Silo"
+ },
+ {
+ "name": "Polahukuna",
+ "pos_x": 59.6875,
+ "pos_y": 84.5,
+ "pos_z": -48.71875,
+ "stations": "Hardy Dock,Clifford Prospect,Vercors Hub,Griffith Dock"
+ },
+ {
+ "name": "Polanque",
+ "pos_x": 121.875,
+ "pos_y": -61.75,
+ "pos_z": -123.9375,
+ "stations": "Binder Platform"
+ },
+ {
+ "name": "Pole",
+ "pos_x": 75.4375,
+ "pos_y": 96.71875,
+ "pos_z": 101.84375,
+ "stations": "Weber Refinery,Ziemkiewicz Platform,Disch Installation,Caryanda Survey"
+ },
+ {
+ "name": "Polecteri",
+ "pos_x": -20.53125,
+ "pos_y": 39.65625,
+ "pos_z": -142.53125,
+ "stations": "Hudson Ring,Ahmed Ring,Huberath Hub,Williams Enterprise,Greenleaf Base"
+ },
+ {
+ "name": "Poleditya",
+ "pos_x": 33.75,
+ "pos_y": -118.90625,
+ "pos_z": 56.15625,
+ "stations": "Backlund Colony"
+ },
+ {
+ "name": "Poletani",
+ "pos_x": 150.5,
+ "pos_y": 2.34375,
+ "pos_z": -18.28125,
+ "stations": "Roddenberry Depot,Clifton Sanctuary,Gerrold Mine"
+ },
+ {
+ "name": "Polevnic",
+ "pos_x": -79.90625,
+ "pos_y": -87.46875,
+ "pos_z": -33.53125,
+ "stations": "Tanner Settlement,Lethem Arsenal,Baird Port,Land Colony"
+ },
+ {
+ "name": "Pollux",
+ "pos_x": 6.71875,
+ "pos_y": 13.71875,
+ "pos_z": -30.125,
+ "stations": "Cook Depot,Naddoddur Survey"
+ },
+ {
+ "name": "Polochans",
+ "pos_x": 14.9375,
+ "pos_y": -90.4375,
+ "pos_z": 39.53125,
+ "stations": "McKean Penal colony,Neujmin Vision,Tanaka Vision"
+ },
+ {
+ "name": "Polohod",
+ "pos_x": 73.375,
+ "pos_y": -30.5625,
+ "pos_z": 67.46875,
+ "stations": "Fermi Settlement"
+ },
+ {
+ "name": "Polontien",
+ "pos_x": -65.46875,
+ "pos_y": 133.28125,
+ "pos_z": 84.96875,
+ "stations": "Linaweaver Dock"
+ },
+ {
+ "name": "Polowar",
+ "pos_x": 155,
+ "pos_y": -37.90625,
+ "pos_z": -7.8125,
+ "stations": "McIntyre Platform,Gaspar de Lemos Orbital,Hollander Base"
+ },
+ {
+ "name": "Polung",
+ "pos_x": 54.4375,
+ "pos_y": 104.59375,
+ "pos_z": -25.4375,
+ "stations": "Kuhn Colony,Poisson's Progress,Joliot-Curie Colony"
+ },
+ {
+ "name": "Polus",
+ "pos_x": 83.8125,
+ "pos_y": 30.90625,
+ "pos_z": 31.5625,
+ "stations": "Wilson Port"
+ },
+ {
+ "name": "Poluskapura",
+ "pos_x": 64.5625,
+ "pos_y": -106.75,
+ "pos_z": -8.59375,
+ "stations": "Molchanov Vision,Fehrenbach Vision,Skjellerup Platform"
+ },
+ {
+ "name": "Pomeche",
+ "pos_x": 56.65625,
+ "pos_y": 82.09375,
+ "pos_z": 3.09375,
+ "stations": "Drexler Colony,Payne Point,Behring Station"
+ },
+ {
+ "name": "Pomeris",
+ "pos_x": -80.34375,
+ "pos_y": -102.46875,
+ "pos_z": -40.875,
+ "stations": "Fermi Port,Wilmore Landing"
+ },
+ {
+ "name": "Pomesci",
+ "pos_x": -47.1875,
+ "pos_y": -196.09375,
+ "pos_z": 21,
+ "stations": "Zubrin Terminal,Nachtigal Bastion,Qureshi Oasis"
+ },
+ {
+ "name": "Pomoco",
+ "pos_x": 25.09375,
+ "pos_y": 25.59375,
+ "pos_z": 144.75,
+ "stations": "Popper Installation,Schilling Plant"
+ },
+ {
+ "name": "Pomotho",
+ "pos_x": -84.40625,
+ "pos_y": -132.90625,
+ "pos_z": 56.46875,
+ "stations": "Kowal Hub,Safdie Dock,MacVicar Works"
+ },
+ {
+ "name": "Ponca Ja",
+ "pos_x": 40.53125,
+ "pos_y": -201.4375,
+ "pos_z": 38.5625,
+ "stations": "McKie Settlement"
+ },
+ {
+ "name": "Poncali",
+ "pos_x": 113.03125,
+ "pos_y": 29.3125,
+ "pos_z": 25.84375,
+ "stations": "de Andrade Mine"
+ },
+ {
+ "name": "Ponchi",
+ "pos_x": 149.84375,
+ "pos_y": -124.90625,
+ "pos_z": 80.25,
+ "stations": "Pajdusakova Dock"
+ },
+ {
+ "name": "Ponchuan",
+ "pos_x": 102.5625,
+ "pos_y": -110.875,
+ "pos_z": 114.25,
+ "stations": "Mori Legacy"
+ },
+ {
+ "name": "Pongkala",
+ "pos_x": -35.90625,
+ "pos_y": 41.6875,
+ "pos_z": -116.46875,
+ "stations": "Aikin City,Simmons Vision,Malzberg Orbital,Brunner Works"
+ },
+ {
+ "name": "Pongmu",
+ "pos_x": 117.5,
+ "pos_y": -32.6875,
+ "pos_z": -2.25,
+ "stations": "Kuipers Beacon"
+ },
+ {
+ "name": "Pongo",
+ "pos_x": -48.71875,
+ "pos_y": -15.71875,
+ "pos_z": 102.46875,
+ "stations": "Antonelli City,Fuchs Survey"
+ },
+ {
+ "name": "Pongs",
+ "pos_x": -39.8125,
+ "pos_y": -180.46875,
+ "pos_z": 37.53125,
+ "stations": "Dilworth Platform"
+ },
+ {
+ "name": "Pontae",
+ "pos_x": 33.46875,
+ "pos_y": 68.84375,
+ "pos_z": 90.46875,
+ "stations": "Manarov Orbital"
+ },
+ {
+ "name": "Pontar",
+ "pos_x": 82.65625,
+ "pos_y": -6.65625,
+ "pos_z": 113.125,
+ "stations": "Sekowski Dock,Walker Holdings,Capek Keep,H. G. Wells Lab"
+ },
+ {
+ "name": "Ponti",
+ "pos_x": 87.90625,
+ "pos_y": -86.6875,
+ "pos_z": 85.375,
+ "stations": "Pickering Settlement"
+ },
+ {
+ "name": "Pontii",
+ "pos_x": -128.28125,
+ "pos_y": 42.1875,
+ "pos_z": 53.96875,
+ "stations": "Gagnan Base,Celsius Orbital"
+ },
+ {
+ "name": "Pontus",
+ "pos_x": -72.25,
+ "pos_y": -18.9375,
+ "pos_z": 33.09375,
+ "stations": "Bolotov Plant,Bohnhoff Keep"
+ },
+ {
+ "name": "Popo Rung",
+ "pos_x": 99.90625,
+ "pos_y": -61.25,
+ "pos_z": 39,
+ "stations": "Melotte City,Levy Plant,Hill Estate,Ryan Plant,Uto Plant"
+ },
+ {
+ "name": "Popocassa",
+ "pos_x": -33.84375,
+ "pos_y": -116.8125,
+ "pos_z": -43.9375,
+ "stations": "Houtman Dock,Citi Legacy,Ore Dock,Linenger Installation,Heaviside Dock,Szameit Hub,Hiroyuki Ring,Quaglia Enterprise,Serrao Enterprise"
+ },
+ {
+ "name": "Popocatepetl",
+ "pos_x": 24.6875,
+ "pos_y": -123.0625,
+ "pos_z": 40.34375,
+ "stations": "Arago Refinery,Reinhold Port"
+ },
+ {
+ "name": "Popoconibo",
+ "pos_x": 5.21875,
+ "pos_y": 31.71875,
+ "pos_z": 70.90625,
+ "stations": "Nelson Dock,Knight Port,Morgan Hub,Lawrence Reach"
+ },
+ {
+ "name": "Popolo",
+ "pos_x": 115.53125,
+ "pos_y": -166.09375,
+ "pos_z": 115.8125,
+ "stations": "Ferguson Platform,Zwicky Port,Anderson Settlement"
+ },
+ {
+ "name": "Popolof",
+ "pos_x": 63.75,
+ "pos_y": -181.46875,
+ "pos_z": -24.59375,
+ "stations": "Alphonsi Point"
+ },
+ {
+ "name": "Popon",
+ "pos_x": -156.78125,
+ "pos_y": 20.84375,
+ "pos_z": -76.75,
+ "stations": "Judson Terminal"
+ },
+ {
+ "name": "Popong O",
+ "pos_x": 127.9375,
+ "pos_y": 62.5625,
+ "pos_z": 100.90625,
+ "stations": "Murray Works,Grandin Landing,Stephenson's Progress,Patrick's Progress"
+ },
+ {
+ "name": "Popongo",
+ "pos_x": 117.6875,
+ "pos_y": -86.5,
+ "pos_z": -125.875,
+ "stations": "Morris Prospect,Powers Landing"
+ },
+ {
+ "name": "Poponna",
+ "pos_x": -62.5,
+ "pos_y": -15.125,
+ "pos_z": -111.71875,
+ "stations": "Polya Vision,Irens Plant,Valdes' Folly"
+ },
+ {
+ "name": "Poponne",
+ "pos_x": 29.625,
+ "pos_y": -134.4375,
+ "pos_z": 29.125,
+ "stations": "Abbot Port,Fan Port,Gould Settlement,Pimi Prospect,Smith's Progress"
+ },
+ {
+ "name": "Poponteno",
+ "pos_x": 51.65625,
+ "pos_y": -67.1875,
+ "pos_z": -92.28125,
+ "stations": "Kaluta Escape"
+ },
+ {
+ "name": "Popontia",
+ "pos_x": 9.0625,
+ "pos_y": -50.15625,
+ "pos_z": -57.125,
+ "stations": "Neff Ring,Ryman Market,Merril Ring"
+ },
+ {
+ "name": "Poqomam",
+ "pos_x": 112.5625,
+ "pos_y": 98.78125,
+ "pos_z": -83.4375,
+ "stations": "Heaviside Dock,Kapp Installation,Wells' Progress"
+ },
+ {
+ "name": "Poqoman",
+ "pos_x": 73.5625,
+ "pos_y": -217.59375,
+ "pos_z": 56.03125,
+ "stations": "al-Din al-Urdi Survey,Lyot Colony,Hay Beacon"
+ },
+ {
+ "name": "Poqomana",
+ "pos_x": -142.90625,
+ "pos_y": 5.15625,
+ "pos_z": 73.3125,
+ "stations": "Cori Terminal,Faris Dock,McCandless Settlement,Fuglesang Refinery,Forest Base"
+ },
+ {
+ "name": "Poqomathi",
+ "pos_x": -98.71875,
+ "pos_y": 23.78125,
+ "pos_z": -71.1875,
+ "stations": "Coulomb Station,Land Camp,Jensen's Progress,Milnor Plant"
+ },
+ {
+ "name": "Poqomawi",
+ "pos_x": 96.78125,
+ "pos_y": -4.46875,
+ "pos_z": 25.90625,
+ "stations": "Hoffmeister Base,Bischoff Hub,Fujimori Terminal,West Dock"
+ },
+ {
+ "name": "Poqomchi",
+ "pos_x": -113.0625,
+ "pos_y": -14,
+ "pos_z": -103.71875,
+ "stations": "Brongniart Settlement,Edgeworth Orbital,So-yeon Survey"
+ },
+ {
+ "name": "Pora",
+ "pos_x": 172.4375,
+ "pos_y": -69.25,
+ "pos_z": 32.34375,
+ "stations": "Lemonnier Vision"
+ },
+ {
+ "name": "Pora Yankas",
+ "pos_x": -82.03125,
+ "pos_y": 97.1875,
+ "pos_z": 96.5,
+ "stations": "Yano Camp,Dirichlet Vision,Barnes Holdings"
+ },
+ {
+ "name": "Poraja",
+ "pos_x": 118.90625,
+ "pos_y": -210.5625,
+ "pos_z": 51,
+ "stations": "Riebe Dock"
+ },
+ {
+ "name": "Porasina",
+ "pos_x": 49.53125,
+ "pos_y": -156.25,
+ "pos_z": -66.5625,
+ "stations": "Honda Vision,Wood's Claim"
+ },
+ {
+ "name": "Porja",
+ "pos_x": 123.03125,
+ "pos_y": 98.3125,
+ "pos_z": -15.25,
+ "stations": "Collins Dock,McHugh Settlement"
+ },
+ {
+ "name": "Portam",
+ "pos_x": 4.84375,
+ "pos_y": 96.4375,
+ "pos_z": 120.75,
+ "stations": "Mieville Prospect,Poncelet Reformatory"
+ },
+ {
+ "name": "Posenae",
+ "pos_x": 57.5,
+ "pos_y": -91.90625,
+ "pos_z": 136.46875,
+ "stations": "De Settlement"
+ },
+ {
+ "name": "Poseno",
+ "pos_x": 81.1875,
+ "pos_y": -125.625,
+ "pos_z": 115.59375,
+ "stations": "Takahashi Port,Chongzhi Hub,Hell Orbital,Christopher Horizons"
+ },
+ {
+ "name": "Posenoi",
+ "pos_x": -47.125,
+ "pos_y": -30.40625,
+ "pos_z": 10,
+ "stations": "Zhigang Plant,Koch Port,Stiegler Bastion,Slade Horizons"
+ },
+ {
+ "name": "Posh",
+ "pos_x": 55.8125,
+ "pos_y": -167.28125,
+ "pos_z": -79.8125,
+ "stations": "Skjellerup Prospect,Plexico Keep,Samos Vision"
+ },
+ {
+ "name": "Potai",
+ "pos_x": 105.75,
+ "pos_y": -175.8125,
+ "pos_z": -52.625,
+ "stations": "Carrasco Installation,Struve Beacon"
+ },
+ {
+ "name": "Potamoi",
+ "pos_x": 81.78125,
+ "pos_y": 0.34375,
+ "pos_z": -10.1875,
+ "stations": "Navigator Landing,Lee Mine"
+ },
+ {
+ "name": "Potar",
+ "pos_x": 154.03125,
+ "pos_y": -102.90625,
+ "pos_z": -60.5625,
+ "stations": "Harvia Vision,Shajn Port,Pook Holdings"
+ },
+ {
+ "name": "Potiguara",
+ "pos_x": -116.875,
+ "pos_y": 15.25,
+ "pos_z": -36.03125,
+ "stations": "Merchiston Dock,Fife Arena"
+ },
+ {
+ "name": "Potilla",
+ "pos_x": 73.34375,
+ "pos_y": -262.65625,
+ "pos_z": -33.6875,
+ "stations": "Adams Beacon"
+ },
+ {
+ "name": "Potilo",
+ "pos_x": -133.09375,
+ "pos_y": 57.9375,
+ "pos_z": -98.5625,
+ "stations": "Bradley Refinery,Bear Settlement"
+ },
+ {
+ "name": "Potres",
+ "pos_x": 107.84375,
+ "pos_y": -141.625,
+ "pos_z": 43.15625,
+ "stations": "Alden Hub,Stein Orbital,Giacconi Dock,Bracewell Gateway"
+ },
+ {
+ "name": "Potri",
+ "pos_x": 17.28125,
+ "pos_y": -123.34375,
+ "pos_z": 96.1875,
+ "stations": "Hassanein Settlement,Shavyrin City,Harvia Vision"
+ },
+ {
+ "name": "Potriambunn",
+ "pos_x": 31.125,
+ "pos_y": -97.5,
+ "pos_z": -20.0625,
+ "stations": "Dupuy de Lome Port,Heinlein Dock"
+ },
+ {
+ "name": "Potrigua",
+ "pos_x": 51.5,
+ "pos_y": -58.25,
+ "pos_z": -44.53125,
+ "stations": "Mitchell City,Arnold Bastion"
+ },
+ {
+ "name": "Potrima",
+ "pos_x": 118.46875,
+ "pos_y": -250.28125,
+ "pos_z": 31.09375,
+ "stations": "Impey Legacy,Korolev's Claim,Dann Plant"
+ },
+ {
+ "name": "Potrimi",
+ "pos_x": 162.90625,
+ "pos_y": -156.625,
+ "pos_z": -35.375,
+ "stations": "Mead Dock,Rittenhouse Ring,Shoemaker Dock,Oramus Terminal,Clark Landing"
+ },
+ {
+ "name": "Potriti",
+ "pos_x": -39.625,
+ "pos_y": -6.59375,
+ "pos_z": 62.59375,
+ "stations": "Hartsfield Plant,Tuan Orbital,Ogden Depot"
+ },
+ {
+ "name": "Poulva",
+ "pos_x": 68.53125,
+ "pos_y": 56.03125,
+ "pos_z": -19.21875,
+ "stations": "Miller Holdings,Vardeman Hub"
+ },
+ {
+ "name": "PPM 41187",
+ "pos_x": -74.125,
+ "pos_y": -1.9375,
+ "pos_z": -23.90625,
+ "stations": "Burnet Port"
+ },
+ {
+ "name": "PPM 5287",
+ "pos_x": 35.9375,
+ "pos_y": -81.875,
+ "pos_z": -47.6875,
+ "stations": "Fulton Depot,Stephenson Terminal"
+ },
+ {
+ "name": "PPM 62114",
+ "pos_x": -110.40625,
+ "pos_y": -6.84375,
+ "pos_z": -11.1875,
+ "stations": "Brillant Reach,Turtledove Arena"
+ },
+ {
+ "name": "Praavapri",
+ "pos_x": 102.71875,
+ "pos_y": -97.1875,
+ "pos_z": 85.625,
+ "stations": "Myasishchev Survey"
+ },
+ {
+ "name": "Prae",
+ "pos_x": 93.03125,
+ "pos_y": 17.78125,
+ "pos_z": -12,
+ "stations": "Thomas Dock,Olsen Platform"
+ },
+ {
+ "name": "Praecipua",
+ "pos_x": 7.4375,
+ "pos_y": 85.5,
+ "pos_z": -40.40625,
+ "stations": "Santos City,Fraser Hub,Harrison Port,Timofeyevich Dock,Ostrander Orbital,Ryman Vista,Cadamosto Depot,Dedekind Hub,Denton Dock,Peano Dock,Plucker Base,Vasquez de Coronado Ring"
+ },
+ {
+ "name": "Praesvatada",
+ "pos_x": 132.53125,
+ "pos_y": -86.5625,
+ "pos_z": 97.75,
+ "stations": "Ings Relay,Edwards Relay"
+ },
+ {
+ "name": "Prahasi",
+ "pos_x": 95.5,
+ "pos_y": -21.9375,
+ "pos_z": -99.71875,
+ "stations": "Valdes Orbital,Fernandez Station,Heinlein Port,Nikitin Terminal,Forstchen City,Tereshkova Depot"
+ },
+ {
+ "name": "Prahlada",
+ "pos_x": -128.3125,
+ "pos_y": -76.28125,
+ "pos_z": 47.84375,
+ "stations": "Virtanen Reach,Fulton Survey"
+ },
+ {
+ "name": "Prahlang",
+ "pos_x": 155.875,
+ "pos_y": -56.46875,
+ "pos_z": -35.46875,
+ "stations": "Reinmuth Landing"
+ },
+ {
+ "name": "Praja",
+ "pos_x": 90.8125,
+ "pos_y": 98.65625,
+ "pos_z": -102.71875,
+ "stations": "Burnham Terminal,Hand Dock,J. G. Ballard City,Anderson Penal colony"
+ },
+ {
+ "name": "Prajan",
+ "pos_x": 56.59375,
+ "pos_y": 139.59375,
+ "pos_z": 10.78125,
+ "stations": "Lebesgue City,Wylie Silo,Mitchell Beacon"
+ },
+ {
+ "name": "Prajapati",
+ "pos_x": -93.1875,
+ "pos_y": 26.375,
+ "pos_z": -119,
+ "stations": "Hopkinson's Folly,Rennie Settlement"
+ },
+ {
+ "name": "Prajau",
+ "pos_x": -20.28125,
+ "pos_y": 15.125,
+ "pos_z": -121.03125,
+ "stations": "Landis Orbital,Wakata Lab,Skolem City,Napier Station"
+ },
+ {
+ "name": "Prana",
+ "pos_x": 21.1875,
+ "pos_y": -165.9375,
+ "pos_z": -52.625,
+ "stations": "Cassini Dock,Otus Works"
+ },
+ {
+ "name": "Priba",
+ "pos_x": 98.9375,
+ "pos_y": -95.5625,
+ "pos_z": 79.9375,
+ "stations": "Chelomey Landing"
+ },
+ {
+ "name": "Pribrog",
+ "pos_x": -100.46875,
+ "pos_y": 76.4375,
+ "pos_z": -39.5625,
+ "stations": "Kregel Mines,Coulomb Terminal,Culpeper Vision,Aitken's Folly,Ziemkiewicz Barracks"
+ },
+ {
+ "name": "Prim",
+ "pos_x": -23.96875,
+ "pos_y": -70.9375,
+ "pos_z": 174.96875,
+ "stations": "Kratman Port,Kagan Survey,Tsunenaga Holdings,Nicolet City,Belyanin Depot"
+ },
+ {
+ "name": "Primi",
+ "pos_x": 135.03125,
+ "pos_y": -143.21875,
+ "pos_z": 89.34375,
+ "stations": "Shimizu Gateway,Flettner Ring,Kiernan Survey,Dreyer Hub"
+ },
+ {
+ "name": "Primpo",
+ "pos_x": -41.65625,
+ "pos_y": -84.28125,
+ "pos_z": -80.5,
+ "stations": "Dana Prospect,Adamson Platform,Butler Depot"
+ },
+ {
+ "name": "Primuni",
+ "pos_x": -91.53125,
+ "pos_y": -148.21875,
+ "pos_z": 122.46875,
+ "stations": "Nobel Horizons,Arnason Port"
+ },
+ {
+ "name": "Princeps",
+ "pos_x": -51.375,
+ "pos_y": 103.28125,
+ "pos_z": 39.03125,
+ "stations": "Cauchy Station,Burgess Enterprise,Fernandes de Queiros Orbital,Godwin Enterprise"
+ },
+ {
+ "name": "Pripai",
+ "pos_x": -41.125,
+ "pos_y": -104.625,
+ "pos_z": 63.375,
+ "stations": "Steinmuller Plant,Anthony Installation"
+ },
+ {
+ "name": "Pripuri",
+ "pos_x": -139.9375,
+ "pos_y": -11.1875,
+ "pos_z": 99.625,
+ "stations": "Merril Platform"
+ },
+ {
+ "name": "Prism",
+ "pos_x": -24.53125,
+ "pos_y": -104,
+ "pos_z": 52,
+ "stations": "Hiram's Anchorage,Viete Colony,Hugh Reformatory"
+ },
+ {
+ "name": "Priva",
+ "pos_x": 78.03125,
+ "pos_y": -50.28125,
+ "pos_z": 28.75,
+ "stations": "Dugan Dock,Aitken Point,Lloyd Wright Settlement,Zindell Landing"
+ },
+ {
+ "name": "Privia",
+ "pos_x": -58.1875,
+ "pos_y": -81,
+ "pos_z": 57.03125,
+ "stations": "Rothman Mine"
+ },
+ {
+ "name": "Priviatem",
+ "pos_x": -21.9375,
+ "pos_y": -119.09375,
+ "pos_z": -11.75,
+ "stations": "Wilhelm Gateway,Smoot Dock,Dowie Depot"
+ },
+ {
+ "name": "Privir",
+ "pos_x": -161.0625,
+ "pos_y": 16.5,
+ "pos_z": -22.875,
+ "stations": "Jones Colony"
+ },
+ {
+ "name": "Privisci",
+ "pos_x": 153.1875,
+ "pos_y": -212,
+ "pos_z": 4.65625,
+ "stations": "Jones Terminal,Axon Works,Kresak Survey"
+ },
+ {
+ "name": "Privithi",
+ "pos_x": 101.8125,
+ "pos_y": -191.71875,
+ "pos_z": 103.25,
+ "stations": "Digges Depot,Gorgani Terminal"
+ },
+ {
+ "name": "Privoro",
+ "pos_x": 66.46875,
+ "pos_y": 62.375,
+ "pos_z": -8.46875,
+ "stations": "Humboldt Mine"
+ },
+ {
+ "name": "Procyon",
+ "pos_x": 6.21875,
+ "pos_y": 2.65625,
+ "pos_z": -9.1875,
+ "stations": "Davy Dock,Greenland's Folly,Cormack Hub,Hardwick Station,Pontes Gateway,Schade Horizons,Mallory Survey,Reightler Station,Sakers Enterprise"
+ },
+ {
+ "name": "Prom",
+ "pos_x": -10.9375,
+ "pos_y": 79.59375,
+ "pos_z": 140.59375,
+ "stations": "Bertin Plant"
+ },
+ {
+ "name": "Prom Du",
+ "pos_x": 2.90625,
+ "pos_y": -1.5625,
+ "pos_z": -120.4375,
+ "stations": "de Sousa Port,Newton Beacon,Hand Colony,Csoma Enterprise,Scheerbart Point"
+ },
+ {
+ "name": "Promba",
+ "pos_x": 98.0625,
+ "pos_y": 34.25,
+ "pos_z": 131.75,
+ "stations": "Maler Dock,Shelley City,Delany Relay,Noether Base"
+ },
+ {
+ "name": "Promes",
+ "pos_x": -4,
+ "pos_y": -193.15625,
+ "pos_z": 95.1875,
+ "stations": "Eddington Vision,Chertovsky Terminal"
+ },
+ {
+ "name": "Proxima",
+ "pos_x": 54.65625,
+ "pos_y": 65.46875,
+ "pos_z": 100.90625,
+ "stations": "Vercors Works,Thomas Kidd Ring"
+ },
+ {
+ "name": "Prthairu",
+ "pos_x": 149.0625,
+ "pos_y": -106.84375,
+ "pos_z": 21.9375,
+ "stations": "Grigorovich Enterprise,Alden City"
+ },
+ {
+ "name": "Prthautas",
+ "pos_x": 142.625,
+ "pos_y": -91,
+ "pos_z": 18.125,
+ "stations": "Penzias Refinery,Brunner Colony,Pearse Survey,Kohoutek Prospect"
+ },
+ {
+ "name": "Prthi",
+ "pos_x": 122.6875,
+ "pos_y": -31.65625,
+ "pos_z": -29.21875,
+ "stations": "Noakes Gateway,Cantor Keep,Kozlov Landing"
+ },
+ {
+ "name": "Prydereti",
+ "pos_x": 67.90625,
+ "pos_y": -113.84375,
+ "pos_z": -49.9375,
+ "stations": "Verrier Orbital,Terry Depot,Karman Enterprise"
+ },
+ {
+ "name": "Psamathe",
+ "pos_x": -76.71875,
+ "pos_y": 7.34375,
+ "pos_z": -28.0625,
+ "stations": "Witt Gateway,Amundsen Hub,Kapp Terminal,Haldeman II Relay"
+ },
+ {
+ "name": "Psi Capricorni",
+ "pos_x": -13.53125,
+ "pos_y": -28.21875,
+ "pos_z": 36.21875,
+ "stations": "Shaw Holdings,So-yeon's Inheritance,Galouye Barracks"
+ },
+ {
+ "name": "Psi Fornacis",
+ "pos_x": 81.0625,
+ "pos_y": -168.21875,
+ "pos_z": -39.5625,
+ "stations": "Wilhelm von Struve Relay"
+ },
+ {
+ "name": "Psi Octantis",
+ "pos_x": 73.90625,
+ "pos_y": -75.46875,
+ "pos_z": 67.8125,
+ "stations": "Gernsback Settlement"
+ },
+ {
+ "name": "Psi Tauri",
+ "pos_x": -19.625,
+ "pos_y": -25.3125,
+ "pos_z": -84.15625,
+ "stations": "Lee Holdings,Pangborn Vision,Stephenson Hub,Knight Terminal,Wohler Orbital,Blackwell Station,Vela Gateway,Anthony Landing"
+ },
+ {
+ "name": "PSPF-LF 2",
+ "pos_x": -4.40625,
+ "pos_y": -17.15625,
+ "pos_z": -15.34375,
+ "stations": "Geston Port,Norton Dock,Salgari Depot"
+ },
+ {
+ "name": "Ptah",
+ "pos_x": 34.15625,
+ "pos_y": -34.71875,
+ "pos_z": -4.5,
+ "stations": "Barcelo Terminal,Lindbohm Dock,Plaskett Arsenal"
+ },
+ {
+ "name": "Pthaa",
+ "pos_x": 123.65625,
+ "pos_y": -178.0625,
+ "pos_z": -5.21875,
+ "stations": "van de Sande Bakhuyzen Vision,Vess Holdings,Qushji's Pride"
+ },
+ {
+ "name": "Pthan Yi",
+ "pos_x": 42.3125,
+ "pos_y": -24.84375,
+ "pos_z": 77.46875,
+ "stations": "Chamitoff Beacon,Hurston Legacy"
+ },
+ {
+ "name": "Pue De",
+ "pos_x": 69.53125,
+ "pos_y": 92.40625,
+ "pos_z": -10.03125,
+ "stations": "Yano Dock"
+ },
+ {
+ "name": "Pue Zhua",
+ "pos_x": -16.4375,
+ "pos_y": -136.40625,
+ "pos_z": -53.3125,
+ "stations": "Aitken Mine"
+ },
+ {
+ "name": "Puelchana",
+ "pos_x": 97.28125,
+ "pos_y": -150.25,
+ "pos_z": -20.53125,
+ "stations": "Aitken Colony,Lindstrand Port,Hansen Port,Akiyama Vision,Frimout Port,Kobayashi Terminal,Cheranovsky Vista,Bode Station,Hollander Orbital,Challis Terminal,Dassault Vision,Maughmer Vision,Thurston Landing,Elbakyan Hub,Busemann Port,Wilhelm von Struve Settlement"
+ },
+ {
+ "name": "Puelchel",
+ "pos_x": -127.875,
+ "pos_y": -55.03125,
+ "pos_z": -65.78125,
+ "stations": "Nicollet Relay,He Reach"
+ },
+ {
+ "name": "Puiki",
+ "pos_x": -91.8125,
+ "pos_y": -43.71875,
+ "pos_z": 0.90625,
+ "stations": "Haise Gateway,Tucker Bastion"
+ },
+ {
+ "name": "Puikiri",
+ "pos_x": -20.96875,
+ "pos_y": -142.375,
+ "pos_z": 65.6875,
+ "stations": "Brouwer Prospect,Nilson Orbital,Burgess Relay,Bolden Holdings"
+ },
+ {
+ "name": "Puikis",
+ "pos_x": -76.46875,
+ "pos_y": 162.9375,
+ "pos_z": -21.53125,
+ "stations": "Samuda Enterprise,Kandel Terminal,Skolem Orbital,Slonczewski Point,Clark's Folly"
+ },
+ {
+ "name": "Puiku",
+ "pos_x": 6.21875,
+ "pos_y": -32.25,
+ "pos_z": 67.59375,
+ "stations": "Dyr Port"
+ },
+ {
+ "name": "Pukkeenat",
+ "pos_x": -65.40625,
+ "pos_y": -8.1875,
+ "pos_z": 167.0625,
+ "stations": "Moon Outpost,Freycinet Installation"
+ },
+ {
+ "name": "Pukkeitha",
+ "pos_x": 58.75,
+ "pos_y": -123.75,
+ "pos_z": -94.59375,
+ "stations": "Foster Hub,McKie Orbital,Wilhelm von Struve Dock,Whitelaw Vision,Roelofs Penal colony"
+ },
+ {
+ "name": "Pukkeitsi",
+ "pos_x": -12.25,
+ "pos_y": 40.75,
+ "pos_z": -146.3125,
+ "stations": "Chiao Hub,Glazkov Terminal,Kwolek Mines,Bixby Relay,Fawcett's Progress"
+ },
+ {
+ "name": "Pulaha",
+ "pos_x": 152.8125,
+ "pos_y": -18.4375,
+ "pos_z": 21.25,
+ "stations": "Barton Settlement,Reed Hub"
+ },
+ {
+ "name": "Pulai",
+ "pos_x": -5.75,
+ "pos_y": 137.53125,
+ "pos_z": 77.90625,
+ "stations": "Amis Prospect,Kozin Stop"
+ },
+ {
+ "name": "Pulangpa",
+ "pos_x": 3.71875,
+ "pos_y": -24.34375,
+ "pos_z": 114.09375,
+ "stations": "Ericsson Orbital,Sadi Carnot Hub,Palmer Terminal"
+ },
+ {
+ "name": "Pulano",
+ "pos_x": 58.21875,
+ "pos_y": -75.90625,
+ "pos_z": 140,
+ "stations": "Pickering Point"
+ },
+ {
+ "name": "Pulara",
+ "pos_x": -46.625,
+ "pos_y": -153.25,
+ "pos_z": 23.5625,
+ "stations": "Ashton Prospect,Hoyle Prospect,Sukhoi Landing,Ordway Horizons"
+ },
+ {
+ "name": "Pularungu",
+ "pos_x": 7.53125,
+ "pos_y": 5.875,
+ "pos_z": -83.03125,
+ "stations": "Berezin Enterprise,Parazynski Point,Citroen Enterprise,Cixin Ring"
+ },
+ {
+ "name": "Pulaya",
+ "pos_x": 134.875,
+ "pos_y": -70.625,
+ "pos_z": 99.28125,
+ "stations": "Maitz Holdings"
+ },
+ {
+ "name": "Punaba",
+ "pos_x": 126.40625,
+ "pos_y": -56.125,
+ "pos_z": -102.65625,
+ "stations": "Harper Vision,O'Donnell Works"
+ },
+ {
+ "name": "Punabon",
+ "pos_x": -60.625,
+ "pos_y": -139.5,
+ "pos_z": 74.5625,
+ "stations": "Rusch Landing,Obruchev Survey,Selberg Base"
+ },
+ {
+ "name": "Punavatii",
+ "pos_x": 70.78125,
+ "pos_y": -119.8125,
+ "pos_z": 77.875,
+ "stations": "Weizsacker Enterprise,Lopez Settlement,Trujillo Point,Schuster Landing,Cayley Enterprise,Heiles Station"
+ },
+ {
+ "name": "Puneith",
+ "pos_x": 10.15625,
+ "pos_y": -49.375,
+ "pos_z": 166.6875,
+ "stations": "Wheelock Port,Papin Works"
+ },
+ {
+ "name": "Punna",
+ "pos_x": -62.96875,
+ "pos_y": -47.0625,
+ "pos_z": 40.25,
+ "stations": "Strekalov Hub,Gauss Works,Nicollier Dock"
+ },
+ {
+ "name": "Punraz",
+ "pos_x": 37.3125,
+ "pos_y": -50.15625,
+ "pos_z": -5.78125,
+ "stations": "Neff Vision,Dashiell Legacy,Almagro Bastion"
+ },
+ {
+ "name": "Punta",
+ "pos_x": -0.875,
+ "pos_y": -168.46875,
+ "pos_z": 7.125,
+ "stations": "Reber Dock,Boyajian Survey,Tolstoi Base"
+ },
+ {
+ "name": "Puntin",
+ "pos_x": 118.09375,
+ "pos_y": 7.09375,
+ "pos_z": -23.3125,
+ "stations": "Martinez Port,Kippax Vision,Vonnegut Mines"
+ },
+ {
+ "name": "Puntinelao",
+ "pos_x": 186.5625,
+ "pos_y": -162.5,
+ "pos_z": 67.125,
+ "stations": "Fabian Port"
+ },
+ {
+ "name": "Punuri",
+ "pos_x": -97.8125,
+ "pos_y": -49.65625,
+ "pos_z": 51.78125,
+ "stations": "Coleman Gateway,Shaw Dock"
+ },
+ {
+ "name": "Punus",
+ "pos_x": 92.875,
+ "pos_y": 58.4375,
+ "pos_z": 95.65625,
+ "stations": "Beliaev Platform"
+ },
+ {
+ "name": "Puppis Sector CQ-Y b1",
+ "pos_x": 53.65625,
+ "pos_y": 3.15625,
+ "pos_z": -46.5,
+ "stations": "The Master of Courage"
+ },
+ {
+ "name": "Purico",
+ "pos_x": 113.9375,
+ "pos_y": 87.625,
+ "pos_z": -60,
+ "stations": "Fremont Landing,Evangelisti Arsenal"
+ },
+ {
+ "name": "Purics",
+ "pos_x": 78.03125,
+ "pos_y": -163.21875,
+ "pos_z": -21,
+ "stations": "Lunney Ring,Bulychev Vision,Wilson Terminal"
+ },
+ {
+ "name": "Purisaz",
+ "pos_x": 96.03125,
+ "pos_y": -10.34375,
+ "pos_z": 46.1875,
+ "stations": "Garratt Enterprise,Schlegel Enterprise,Gaiman Beacon,Lichtenberg Relay,Chaudhary Gateway"
+ },
+ {
+ "name": "Purubalumbo",
+ "pos_x": -17.875,
+ "pos_y": -98.46875,
+ "pos_z": -133,
+ "stations": "Svavarsson Dock,Buchli Settlement"
+ },
+ {
+ "name": "Purubo",
+ "pos_x": 12.625,
+ "pos_y": 45.5,
+ "pos_z": 140.125,
+ "stations": "Lee Terminal,Davy Mines"
+ },
+ {
+ "name": "Purui",
+ "pos_x": -32.46875,
+ "pos_y": 104.75,
+ "pos_z": 29.78125,
+ "stations": "Herbert Survey,Cady Landing"
+ },
+ {
+ "name": "Purui Hari",
+ "pos_x": -13.09375,
+ "pos_y": 80.34375,
+ "pos_z": -69.03125,
+ "stations": "Duke Colony"
+ },
+ {
+ "name": "Purui Xuang",
+ "pos_x": 81.21875,
+ "pos_y": -35.34375,
+ "pos_z": 47,
+ "stations": "Piper Refinery"
+ },
+ {
+ "name": "Purukuna",
+ "pos_x": 151.3125,
+ "pos_y": 40.46875,
+ "pos_z": -81.84375,
+ "stations": "Pettit Base,Buckland Settlement"
+ },
+ {
+ "name": "Puruma",
+ "pos_x": 18.875,
+ "pos_y": -146.9375,
+ "pos_z": 134.96875,
+ "stations": "Unsold Prospect,Webb Observatory,Folland Station"
+ },
+ {
+ "name": "Purungaitha",
+ "pos_x": -79.84375,
+ "pos_y": 98.9375,
+ "pos_z": -112.1875,
+ "stations": "Alexander Terminal,Makeev Horizons,Rustah Horizons,Back Legacy"
+ },
+ {
+ "name": "Purungarang",
+ "pos_x": -34.84375,
+ "pos_y": 28.125,
+ "pos_z": 110.8125,
+ "stations": "Babbage Terminal,Newman Installation"
+ },
+ {
+ "name": "Purungga",
+ "pos_x": 24,
+ "pos_y": -77.28125,
+ "pos_z": 108.90625,
+ "stations": "McKay Terminal,Adams Beacon,Koontz Barracks,Bentham Vision"
+ },
+ {
+ "name": "Purungkat",
+ "pos_x": 20.625,
+ "pos_y": 31.125,
+ "pos_z": -162.46875,
+ "stations": "Howard Dock,Simonyi Port,Sturt Settlement"
+ },
+ {
+ "name": "Purungurawn",
+ "pos_x": 115.625,
+ "pos_y": -152.875,
+ "pos_z": 75.375,
+ "stations": "Borrelly Hub"
+ },
+ {
+ "name": "Purunsi",
+ "pos_x": 48.75,
+ "pos_y": 18.78125,
+ "pos_z": 112.15625,
+ "stations": "Taylor Station,Ohm Enterprise,Burgess Barracks,Zewail Terminal,Furukawa Hub,Konscak Enterprise,Rontgen Dock,Alexandria Hub,Bailey's Claim,Lazarev Dock,Bottego's Inheritance"
+ },
+ {
+ "name": "Purusha",
+ "pos_x": -60.8125,
+ "pos_y": 68.3125,
+ "pos_z": 74.96875,
+ "stations": "Wisniewski-Snerg Orbital,Baydukov Terminal,Norman Vision,Stableford Base"
+ },
+ {
+ "name": "Purut",
+ "pos_x": -63.15625,
+ "pos_y": -62.03125,
+ "pos_z": -90.5,
+ "stations": "Liouville Plant,Macan Dock,Tshang Landing,Alvares Observatory"
+ },
+ {
+ "name": "Pushis",
+ "pos_x": -10.03125,
+ "pos_y": 61.78125,
+ "pos_z": 120.3125,
+ "stations": "Al-Khalili Vision,Kregel Enterprise,Ivanishin Colony,Sutcliffe Escape"
+ },
+ {
+ "name": "Puskabui",
+ "pos_x": 54.125,
+ "pos_y": 62.375,
+ "pos_z": 6.4375,
+ "stations": "Rodrigues Orbital,Bates Prospect,Fowler Works"
+ },
+ {
+ "name": "Puskas",
+ "pos_x": 93.5625,
+ "pos_y": -36.6875,
+ "pos_z": -122.21875,
+ "stations": "Atwater Orbital,Jemison Hub,Diesel Enterprise,Carver Keep"
+ },
+ {
+ "name": "Putama",
+ "pos_x": 80.84375,
+ "pos_y": -17,
+ "pos_z": -45.96875,
+ "stations": "Levi-Civita Ring,Swift Station,Watts Gateway"
+ },
+ {
+ "name": "Putamasin",
+ "pos_x": 37.21875,
+ "pos_y": -76.375,
+ "pos_z": 21.28125,
+ "stations": "Weil Orbital,Corbusier Arsenal,Hartog Terminal,Piccard Observatory,Baffin Gateway"
+ },
+ {
+ "name": "Putana",
+ "pos_x": 116.59375,
+ "pos_y": 18,
+ "pos_z": -75.3125,
+ "stations": "Foucault Ring,Cabana Hub,Hurston Landing"
+ },
+ {
+ "name": "Putanitu",
+ "pos_x": 163.78125,
+ "pos_y": -59.6875,
+ "pos_z": 36.9375,
+ "stations": "Bracewell City,Piano Port,Dorsey Works,Hiroyuki Prospect,Hanke-Woods Terminal,Gerrold Landing"
+ },
+ {
+ "name": "Putas",
+ "pos_x": 159.9375,
+ "pos_y": -94.71875,
+ "pos_z": -45.25,
+ "stations": "Ryan Port,Delaunay Orbital"
+ },
+ {
+ "name": "PW Hydrae",
+ "pos_x": 65,
+ "pos_y": 38.5625,
+ "pos_z": -6.21875,
+ "stations": "Freycinet Station,Jiushao Depot,Dummer Vision,Wandrei Station,Nixon Station,Sleator City,Coelho Port,Sakers Station,Fourier Port"
+ },
+ {
+ "name": "Pwyll",
+ "pos_x": 33.34375,
+ "pos_y": -4.375,
+ "pos_z": 5.78125,
+ "stations": "Northrop Refinery,Tanner Holdings,Papin Orbital,Mattingly Port"
+ },
+ {
+ "name": "Pyemma",
+ "pos_x": -80.53125,
+ "pos_y": -12.625,
+ "pos_z": -121.375,
+ "stations": "Beadle Port,Adamson Mines,Hardy Base,White Station,Steakley Settlement"
+ },
+ {
+ "name": "Pyemmairre",
+ "pos_x": 2.84375,
+ "pos_y": -125.6875,
+ "pos_z": 6.8125,
+ "stations": "Flettner Horizons"
+ },
+ {
+ "name": "Pyemmamayo",
+ "pos_x": 57.46875,
+ "pos_y": 82.3125,
+ "pos_z": 100.625,
+ "stations": "Rice Dock,Lamarr Terminal"
+ },
+ {
+ "name": "Pyemmar",
+ "pos_x": 122.5,
+ "pos_y": 46.65625,
+ "pos_z": 24.375,
+ "stations": "Kotov Works"
+ },
+ {
+ "name": "Pyemmatsety",
+ "pos_x": 105.96875,
+ "pos_y": -20.875,
+ "pos_z": -22.21875,
+ "stations": "Haller Orbital,McKay Settlement,Torricelli Station,Cady Base"
+ },
+ {
+ "name": "Pyemsito",
+ "pos_x": -39.90625,
+ "pos_y": 47.78125,
+ "pos_z": 40.4375,
+ "stations": "Sanger Hub,Cramer Survey"
+ },
+ {
+ "name": "Pyemsitoq",
+ "pos_x": 72.03125,
+ "pos_y": 71.625,
+ "pos_z": -88.40625,
+ "stations": "Thiele Holdings,Lopez de Haro Relay"
+ },
+ {
+ "name": "Pyritapiyar",
+ "pos_x": -29.0625,
+ "pos_y": -18.8125,
+ "pos_z": -128.46875,
+ "stations": "Morgan Dock,Herreshoff Keep,Vinogradov Mine"
+ },
+ {
+ "name": "Pyrrha",
+ "pos_x": -9529.59375,
+ "pos_y": -902.03125,
+ "pos_z": 19826.375,
+ "stations": "Tsiolkovskiy Horizon"
+ },
+ {
+ "name": "Pytheas",
+ "pos_x": -9524.53125,
+ "pos_y": -909.0625,
+ "pos_z": 19793.28125,
+ "stations": "Hipparque - Cartographers' University"
+ },
+ {
+ "name": "Q Scorpii",
+ "pos_x": 25.5625,
+ "pos_y": -11.90625,
+ "pos_z": 163.375,
+ "stations": "Laing Settlement,Judson Hub,Malocello Orbital"
+ },
+ {
+ "name": "q Velorum",
+ "pos_x": 98.8125,
+ "pos_y": 20.875,
+ "pos_z": 8.28125,
+ "stations": "Larson Lab,Bain Landing"
+ },
+ {
+ "name": "Q'eqchi",
+ "pos_x": -42.8125,
+ "pos_y": -98.8125,
+ "pos_z": 25.46875,
+ "stations": "Weston Landing"
+ },
+ {
+ "name": "Q'eqchilir",
+ "pos_x": -92.25,
+ "pos_y": 18.53125,
+ "pos_z": -119.1875,
+ "stations": "Moran Terminal,Bulgarin Landing,Russ Port,Priest's Claim,Johnson Vision"
+ },
+ {
+ "name": "Q'eqchit",
+ "pos_x": -33.21875,
+ "pos_y": -44.15625,
+ "pos_z": 107.03125,
+ "stations": "Blair's Folly,Spielberg Installation"
+ },
+ {
+ "name": "Q'ero",
+ "pos_x": 16.125,
+ "pos_y": -17.21875,
+ "pos_z": 173.03125,
+ "stations": "Baudin Landing"
+ },
+ {
+ "name": "q1 Eridani",
+ "pos_x": 25.71875,
+ "pos_y": -50.15625,
+ "pos_z": 7.4375,
+ "stations": "Windt Terminal,Roddenberry Installation,Ziemkiewicz Terminal,Brin Vision"
+ },
+ {
+ "name": "Qa'wakana",
+ "pos_x": -17.65625,
+ "pos_y": -19.125,
+ "pos_z": 61.78125,
+ "stations": "Carpini Terminal,al-Haytham Terminal,Sargent Installation,Beaufoy Hub,Farrukh Terminal,Cseszneky Settlement,Tall Dock,Everest Enterprise,Fairbairn Escape,Warner Landing"
+ },
+ {
+ "name": "Qa'wakwanga",
+ "pos_x": 56.4375,
+ "pos_y": -204.71875,
+ "pos_z": 73.03125,
+ "stations": "Prandtl Port,Kepler Settlement"
+ },
+ {
+ "name": "Qaha Witoto",
+ "pos_x": -60.53125,
+ "pos_y": -25.4375,
+ "pos_z": 100.5,
+ "stations": "Deere Gateway,Eskridge Depot,Chandler Beacon,Laumer Base"
+ },
+ {
+ "name": "Qahamoano",
+ "pos_x": 119.15625,
+ "pos_y": 108.59375,
+ "pos_z": 35.71875,
+ "stations": "Bloch Orbital,Jeschke City,Kennicott Enterprise,Wyndham Gateway"
+ },
+ {
+ "name": "Qahastis",
+ "pos_x": 74.75,
+ "pos_y": -181.5,
+ "pos_z": 119.90625,
+ "stations": "Russell Installation,Weaver Landing,Fernandes Penal colony,Jones Terminal"
+ },
+ {
+ "name": "Qahat",
+ "pos_x": -136.53125,
+ "pos_y": 19.84375,
+ "pos_z": -140.90625,
+ "stations": "Saavedra Port,Macdonald's Progress"
+ },
+ {
+ "name": "Qahatini",
+ "pos_x": 65.59375,
+ "pos_y": -42.875,
+ "pos_z": 77.375,
+ "stations": "Nicollet Settlement"
+ },
+ {
+ "name": "Qama",
+ "pos_x": -86.3125,
+ "pos_y": -16.96875,
+ "pos_z": -34.875,
+ "stations": "Thurston Ring,Serrao Vision,Wohler Observatory,Drzewiecki Hub"
+ },
+ {
+ "name": "Qamadi",
+ "pos_x": -58.8125,
+ "pos_y": 80.5,
+ "pos_z": 38.46875,
+ "stations": "Fuca Stop,Lebesgue Camp"
+ },
+ {
+ "name": "Qaratay",
+ "pos_x": 161.4375,
+ "pos_y": -103.4375,
+ "pos_z": 116.9375,
+ "stations": "Ziemianski Point,Barlowe Dock,Polikarpov Prospect,Deutsch Platform"
+ },
+ {
+ "name": "Qarato",
+ "pos_x": 7.5,
+ "pos_y": -58.78125,
+ "pos_z": 18,
+ "stations": "Drexler Dock,Tsunenaga's Folly,Worlidge Hangar"
+ },
+ {
+ "name": "Qawamita",
+ "pos_x": -99.375,
+ "pos_y": -90.1875,
+ "pos_z": -102.25,
+ "stations": "Burgess Colony,He Platform,Morrison Beacon"
+ },
+ {
+ "name": "Qawar",
+ "pos_x": 82.25,
+ "pos_y": -60.96875,
+ "pos_z": -64.84375,
+ "stations": "Scully-Power Camp,Carr Platform,Carstensz Holdings"
+ },
+ {
+ "name": "Qawasing",
+ "pos_x": -107.75,
+ "pos_y": 9.53125,
+ "pos_z": -119.8125,
+ "stations": "Cavalieri Landing"
+ },
+ {
+ "name": "Qetesh",
+ "pos_x": 125.125,
+ "pos_y": -161.78125,
+ "pos_z": 47.15625,
+ "stations": "Baynes Terminal,Siegel Relay,Whipple Vision,Phillips' Progress,Jeury Survey,Wilhelm Horizons"
+ },
+ {
+ "name": "Qi Gongzi",
+ "pos_x": 161.71875,
+ "pos_y": -11.6875,
+ "pos_z": 61,
+ "stations": "Worlidge Hub,Chadwick Silo,Chebyshev Laboratory"
+ },
+ {
+ "name": "Qi Lieh",
+ "pos_x": 30.40625,
+ "pos_y": 70.3125,
+ "pos_z": -27.3125,
+ "stations": "Humboldt Survey,Garan Mines,Weiss Enterprise"
+ },
+ {
+ "name": "Qi Lisu",
+ "pos_x": 105.65625,
+ "pos_y": 6.1875,
+ "pos_z": -24.6875,
+ "stations": "Hausdorff City,Merril Oasis"
+ },
+ {
+ "name": "Qi Yi",
+ "pos_x": -127,
+ "pos_y": -56.34375,
+ "pos_z": -45.65625,
+ "stations": "Low Hub,Coulomb Port,Gidzenko City,Sawyer Depot,Pashin Reach"
+ },
+ {
+ "name": "Qi Yomisii",
+ "pos_x": -45.5,
+ "pos_y": -38.1875,
+ "pos_z": -91.15625,
+ "stations": "Weitz Orbital,Seddon Terminal,Aitken Settlement,King Point,Berners-Lee Holdings"
+ },
+ {
+ "name": "Qi Yum Crua",
+ "pos_x": 123.6875,
+ "pos_y": 27.46875,
+ "pos_z": 92.78125,
+ "stations": "Bierce Orbital,Hennepin Dock,Kratman City,Wiener Arsenal"
+ },
+ {
+ "name": "Qi Yun Cech",
+ "pos_x": 175.59375,
+ "pos_y": -155.625,
+ "pos_z": 21.65625,
+ "stations": "Calatrava Colony,Littrow Station,Brundage Orbital,Bridger Legacy"
+ },
+ {
+ "name": "Qin Tsu",
+ "pos_x": -11.25,
+ "pos_y": 85.71875,
+ "pos_z": -106.0625,
+ "stations": "Lorrah Relay,VanderMeer Settlement,Pinto Hangar"
+ },
+ {
+ "name": "Qin Yane",
+ "pos_x": 200.4375,
+ "pos_y": -117.53125,
+ "pos_z": -13,
+ "stations": "Bonestell Station,Nadiradze Vision,Halley Station,Mackenzie Landing,Helin Installation,Nobel Base"
+ },
+ {
+ "name": "Qinganu",
+ "pos_x": 149.5,
+ "pos_y": 12.625,
+ "pos_z": -99.75,
+ "stations": "Lasswitz Plant,Schiltberger Settlement,Foden Hub"
+ },
+ {
+ "name": "QQ Pegasi",
+ "pos_x": -59.625,
+ "pos_y": -56.9375,
+ "pos_z": 13.8125,
+ "stations": "Buchli Station,Kondratyev City"
+ },
+ {
+ "name": "Quaaya",
+ "pos_x": 60.75,
+ "pos_y": -144.90625,
+ "pos_z": -98.09375,
+ "stations": "Chadwick Port,Conti Arsenal,Taylor Gateway,Kobayashi Orbital,Russell Lab"
+ },
+ {
+ "name": "Quaaybuwala",
+ "pos_x": 110.65625,
+ "pos_y": -73.125,
+ "pos_z": -37.78125,
+ "stations": "Roelofs Hub,Marth Dock,Pu Station,Friedrich Peters Dock,Clark's Pride"
+ },
+ {
+ "name": "Quaaybuwan",
+ "pos_x": 133.21875,
+ "pos_y": 111.90625,
+ "pos_z": 102.34375,
+ "stations": "Blair Relay"
+ },
+ {
+ "name": "Quambo",
+ "pos_x": -51.6875,
+ "pos_y": 70.46875,
+ "pos_z": -110.65625,
+ "stations": "Macedo's Progress,Simpson Terminal,Musa al-Khwarizmi Orbital"
+ },
+ {
+ "name": "Quamta",
+ "pos_x": 43.5,
+ "pos_y": -139.34375,
+ "pos_z": 1.59375,
+ "stations": "Sitterly Relay,Otomo Port,Tully Survey"
+ },
+ {
+ "name": "Quamte",
+ "pos_x": 153.28125,
+ "pos_y": -72.96875,
+ "pos_z": 18.09375,
+ "stations": "Secchi Dock"
+ },
+ {
+ "name": "Quan Gurus",
+ "pos_x": 11.28125,
+ "pos_y": -13.8125,
+ "pos_z": 90.28125,
+ "stations": "Russell Dock,Heaviside Gateway,Fernandes Terminal,Serrao Settlement"
+ },
+ {
+ "name": "Quaoar",
+ "pos_x": 54.6875,
+ "pos_y": 157,
+ "pos_z": 26.65625,
+ "stations": "Ferguson Vision,Sarich City,Gurragchaa Survey,Diesel Enterprise"
+ },
+ {
+ "name": "Quapa",
+ "pos_x": 6.3125,
+ "pos_y": -34.40625,
+ "pos_z": 32.59375,
+ "stations": "Grabe Dock,Ashton Bastion,Carrier Ring,Matteucci Terminal"
+ },
+ {
+ "name": "Quapani",
+ "pos_x": 4.25,
+ "pos_y": -168.53125,
+ "pos_z": 71.03125,
+ "stations": "Mikulin Point"
+ },
+ {
+ "name": "Quaqu",
+ "pos_x": -17.71875,
+ "pos_y": 52.5,
+ "pos_z": -132.4375,
+ "stations": "Wohler Hangar,Thomas Vision"
+ },
+ {
+ "name": "Quariti",
+ "pos_x": 96.625,
+ "pos_y": 5.46875,
+ "pos_z": 6.6875,
+ "stations": "Malerba Station,Kimbrough Enterprise,Roosa Hub,Bering Barracks,Auld Beacon"
+ },
+ {
+ "name": "Quator",
+ "pos_x": 71.625,
+ "pos_y": 47.75,
+ "pos_z": 64.8125,
+ "stations": "Weber Settlement,Quator Station"
+ },
+ {
+ "name": "Qudshep",
+ "pos_x": 57.65625,
+ "pos_y": -81.4375,
+ "pos_z": 104.78125,
+ "stations": "Sagan Plant,Baillaud City,Whitcomb Platform,Cabot Reach"
+ },
+ {
+ "name": "Queche",
+ "pos_x": -92.125,
+ "pos_y": 57.21875,
+ "pos_z": -27.53125,
+ "stations": "Blaschke Refinery,Darboux's Inheritance"
+ },
+ {
+ "name": "Quechi",
+ "pos_x": 162.3125,
+ "pos_y": -61.6875,
+ "pos_z": 74.6875,
+ "stations": "Takamizawa Mine,Mobius Landing,Aitken Barracks"
+ },
+ {
+ "name": "Quechua",
+ "pos_x": 52.09375,
+ "pos_y": -112.09375,
+ "pos_z": -40.78125,
+ "stations": "Crown Ring"
+ },
+ {
+ "name": "Quiablinja",
+ "pos_x": 86.5625,
+ "pos_y": -82.75,
+ "pos_z": 82.40625,
+ "stations": "Linge Point,Vess Landing,al-Kashi Dock"
+ },
+ {
+ "name": "Quiara",
+ "pos_x": -39.6875,
+ "pos_y": 116.1875,
+ "pos_z": 58.90625,
+ "stations": "Platt Prison,Vonnegut Prospect,Humphreys Platform"
+ },
+ {
+ "name": "Quiate",
+ "pos_x": 94.125,
+ "pos_y": -133.15625,
+ "pos_z": 15.40625,
+ "stations": "Oberth Station"
+ },
+ {
+ "name": "Quikil",
+ "pos_x": 51.03125,
+ "pos_y": -5.75,
+ "pos_z": -53.4375,
+ "stations": "Fidalgo Base,Lenoir Enterprise,Crown Station,Stebler Enterprise,la Cosa Beacon"
+ },
+ {
+ "name": "Quikindji",
+ "pos_x": -89.6875,
+ "pos_y": -31.21875,
+ "pos_z": 19.71875,
+ "stations": "Akiyama Platform,Murphy Works,Wolf Hangar"
+ },
+ {
+ "name": "Quikinnaqu",
+ "pos_x": 89.09375,
+ "pos_y": 13.40625,
+ "pos_z": 110.625,
+ "stations": "Ivins Dock,Baker Plant,DeLucas Platform"
+ },
+ {
+ "name": "Quikudi",
+ "pos_x": -35.65625,
+ "pos_y": -40.40625,
+ "pos_z": 72.4375,
+ "stations": "Frobisher Penal colony"
+ },
+ {
+ "name": "Quikuruba",
+ "pos_x": 188.875,
+ "pos_y": -173.5,
+ "pos_z": 2.03125,
+ "stations": "Ernst Vision,Back Barracks"
+ },
+ {
+ "name": "Quile",
+ "pos_x": 69.84375,
+ "pos_y": -206.3125,
+ "pos_z": 71.96875,
+ "stations": "Abetti Port,Weizsacker Port,Hartmann Hub,Messerschmitt Point,Ludwig Struve Point,Humphreys Landing,Nakamura Settlement,Gibson Enterprise,Hatanaka Port"
+ },
+ {
+ "name": "Quillaente",
+ "pos_x": 98.375,
+ "pos_y": -184.21875,
+ "pos_z": 138.15625,
+ "stations": "Mukai Hub,Coggia Gateway,Wells Enterprise"
+ },
+ {
+ "name": "Quilmen",
+ "pos_x": -77.71875,
+ "pos_y": 117.15625,
+ "pos_z": 80.21875,
+ "stations": "Wrangell Landing,McMonagle Landing,Borisenko Relay"
+ },
+ {
+ "name": "Quilmes",
+ "pos_x": 8.5625,
+ "pos_y": -28.4375,
+ "pos_z": 40.3125,
+ "stations": "Bethe Installation,Wescott Vision"
+ },
+ {
+ "name": "Quince",
+ "pos_x": 57.375,
+ "pos_y": 341.375,
+ "pos_z": 333,
+ "stations": "Millerport,Jeffries High,Dahm Exchange"
+ },
+ {
+ "name": "Quiness",
+ "pos_x": -46.46875,
+ "pos_y": 37.78125,
+ "pos_z": -24.9375,
+ "stations": "Lockyer's Rest"
+ },
+ {
+ "name": "Quitakiana",
+ "pos_x": 59.84375,
+ "pos_y": -144.75,
+ "pos_z": -31.3125,
+ "stations": "Boyajian Station"
+ },
+ {
+ "name": "Quivira",
+ "pos_x": 33.375,
+ "pos_y": -112.875,
+ "pos_z": 1.65625,
+ "stations": "Baily Penal colony,Godel Dock,Lippisch Vision,Stapledon Dock,Brendan Gateway"
+ },
+ {
+ "name": "Ququve",
+ "pos_x": -44.1875,
+ "pos_y": -17.125,
+ "pos_z": -60.1875,
+ "stations": "Peters Holm,Smirnova Relay"
+ },
+ {
+ "name": "R Doradus",
+ "pos_x": 157.375,
+ "pos_y": -128.96875,
+ "pos_z": 6.90625,
+ "stations": "Arnold Market,Fabian Terminal"
+ },
+ {
+ "name": "Ra",
+ "pos_x": -20.84375,
+ "pos_y": -43.9375,
+ "pos_z": 22.65625,
+ "stations": "Bohr Survey,Burkin Base,LeConte Dock,Kazantsev Dock,Coelho Ring,Kandrup Beacon,Ellison Orbital,Lehtonen Hub,Taine Landing,Roemer Landing,Gohar Gateway,Delbruck Settlement,Chu Silo"
+ },
+ {
+ "name": "Ra' Takakhan",
+ "pos_x": -9530.375,
+ "pos_y": -911,
+ "pos_z": 19840.9375,
+ "stations": "Muir Survey"
+ },
+ {
+ "name": "Raba",
+ "pos_x": 68.53125,
+ "pos_y": -132.28125,
+ "pos_z": 73.25,
+ "stations": "Whittle Mines,Vaisala Survey"
+ },
+ {
+ "name": "Rabakama",
+ "pos_x": 147.125,
+ "pos_y": 26.0625,
+ "pos_z": 54.625,
+ "stations": "McIntyre Gateway,Kress Station,Nesvadba Platform,Lloyd Base,Liebig Observatory"
+ },
+ {
+ "name": "Rabakshany",
+ "pos_x": 83.28125,
+ "pos_y": -78.875,
+ "pos_z": 31.59375,
+ "stations": "Armero Port,Lindblad Station,Erdos Horizons,van Rhijn Orbital,Messerschmitt Orbital,Dixon Station,Potocnik Hub,Besonders Plant,Al Sufi Orbital,Merchiston Settlement"
+ },
+ {
+ "name": "Rabal",
+ "pos_x": -127.8125,
+ "pos_y": -112.53125,
+ "pos_z": 19.96875,
+ "stations": "Davis Station,Pratchett Orbital,Gresh Installation,Kozlov Laboratory"
+ },
+ {
+ "name": "Rabastyane",
+ "pos_x": -43.3125,
+ "pos_y": -42.125,
+ "pos_z": 85.53125,
+ "stations": "Locke's Claim"
+ },
+ {
+ "name": "Rabatha",
+ "pos_x": 10.75,
+ "pos_y": -153.90625,
+ "pos_z": -22.8125,
+ "stations": "Herbert's Inheritance,Adams Colony,Lambert Landing"
+ },
+ {
+ "name": "Rabay",
+ "pos_x": -73.875,
+ "pos_y": 42.40625,
+ "pos_z": 38.15625,
+ "stations": "Raleigh Port,Marques Port,Huberath Vista,Stevens Enterprise,Windt Legacy,Sabine Orbital,Gardner Market,Cabrera Terminal,Kuo Port,Clute Base,Utley Terminal,Ostrander Depot"
+ },
+ {
+ "name": "Rabh",
+ "pos_x": 101.03125,
+ "pos_y": -64.84375,
+ "pos_z": -13.875,
+ "stations": "Muller Terminal,Friedman Terminal,Goldschmidt Terminal,Bass Prospect,Baade Survey,Dahm Vision,Reinmuth Port,Campbell Plant"
+ },
+ {
+ "name": "Radegast",
+ "pos_x": -18,
+ "pos_y": 32.375,
+ "pos_z": 43.46875,
+ "stations": "Waldeck Platform"
+ },
+ {
+ "name": "Radgud",
+ "pos_x": -129.0625,
+ "pos_y": -30.21875,
+ "pos_z": 4.9375,
+ "stations": "Bridges Terminal,Khan Enterprise"
+ },
+ {
+ "name": "Radiansan",
+ "pos_x": 55.875,
+ "pos_y": 38.875,
+ "pos_z": -34.28125,
+ "stations": "Chang-Diaz Enterprise,Arber Installation,Hutton Station"
+ },
+ {
+ "name": "Radican",
+ "pos_x": 101.6875,
+ "pos_y": 16.25,
+ "pos_z": -19.8125,
+ "stations": "Kimbrough Outpost"
+ },
+ {
+ "name": "Radigast",
+ "pos_x": 116.125,
+ "pos_y": 19.3125,
+ "pos_z": 76.90625,
+ "stations": "Smeaton Prospect,Goeschke Colony,Fontenay Bastion"
+ },
+ {
+ "name": "Radimichs",
+ "pos_x": -45.03125,
+ "pos_y": -96.8125,
+ "pos_z": 34.59375,
+ "stations": "Mitchell Hub,Sakers Hangar,Burroughs' Folly"
+ },
+ {
+ "name": "Ragana",
+ "pos_x": 142.875,
+ "pos_y": -208.4375,
+ "pos_z": 38.78125,
+ "stations": "Sugie Station,Dornier Orbital,Coddington Point"
+ },
+ {
+ "name": "Ragapajo",
+ "pos_x": 45.90625,
+ "pos_y": 32.03125,
+ "pos_z": -64.03125,
+ "stations": "Kazantsev Terminal,Vinci Orbital,Hadfield Penal colony"
+ },
+ {
+ "name": "Ragnorak",
+ "pos_x": -32.3125,
+ "pos_y": 74.3125,
+ "pos_z": 64.25,
+ "stations": "Morgan Orbital,Schlegel Landing,Hadfield Depot,Poindexter Colony,Vesalius Refinery"
+ },
+ {
+ "name": "Raguanan",
+ "pos_x": 60.5625,
+ "pos_y": -222.59375,
+ "pos_z": 38.9375,
+ "stations": "Trimble Colony"
+ },
+ {
+ "name": "Raguti",
+ "pos_x": -90,
+ "pos_y": -10.21875,
+ "pos_z": -97.25,
+ "stations": "Baraniecki Hub,Phillpotts Dock,Bellamy Installation,Warner Dock"
+ },
+ {
+ "name": "Raguvii",
+ "pos_x": 2.5,
+ "pos_y": 32.59375,
+ "pos_z": 55.125,
+ "stations": "Britnev Platform"
+ },
+ {
+ "name": "Rahu",
+ "pos_x": -43.78125,
+ "pos_y": 62.4375,
+ "pos_z": -0.25,
+ "stations": "Acharya Station,Kelly Penal colony"
+ },
+ {
+ "name": "Raidal",
+ "pos_x": -14.28125,
+ "pos_y": -61.96875,
+ "pos_z": -150.8125,
+ "stations": "Gagarin Stop,Bellamy Settlement"
+ },
+ {
+ "name": "Raidar",
+ "pos_x": 58.125,
+ "pos_y": 16.21875,
+ "pos_z": 99.96875,
+ "stations": "Gelfand's Exile"
+ },
+ {
+ "name": "Raidenwitha",
+ "pos_x": 127.96875,
+ "pos_y": -99.84375,
+ "pos_z": 28.34375,
+ "stations": "West Holdings,Brunner Settlement,Biesbroeck Vision"
+ },
+ {
+ "name": "Raijankas",
+ "pos_x": -120.1875,
+ "pos_y": -3.53125,
+ "pos_z": -92.65625,
+ "stations": "Levinson Colony"
+ },
+ {
+ "name": "Raijin",
+ "pos_x": 121.46875,
+ "pos_y": -68.875,
+ "pos_z": -0.375,
+ "stations": "May Ring"
+ },
+ {
+ "name": "Raiju",
+ "pos_x": 75.125,
+ "pos_y": 100.46875,
+ "pos_z": -53.65625,
+ "stations": "Hawley Prospect,Fincke Installation,Schroeder Horizons"
+ },
+ {
+ "name": "Raijuhan",
+ "pos_x": -59.40625,
+ "pos_y": -20.6875,
+ "pos_z": -138.5625,
+ "stations": "Gann Mines"
+ },
+ {
+ "name": "Raijuhan Yi",
+ "pos_x": 17.65625,
+ "pos_y": -235.9375,
+ "pos_z": 69.90625,
+ "stations": "Mattei Hub,Hansen Vision,Gutierrez Survey,Stiegler Survey"
+ },
+ {
+ "name": "Raijuhty",
+ "pos_x": 67.65625,
+ "pos_y": -32.875,
+ "pos_z": -74.375,
+ "stations": "Yang Dock"
+ },
+ {
+ "name": "Raijunab",
+ "pos_x": 116.6875,
+ "pos_y": 29.1875,
+ "pos_z": 27.90625,
+ "stations": "Ham Platform,Duckworth Ring,Moisuc Terminal"
+ },
+ {
+ "name": "Rain",
+ "pos_x": -12.03125,
+ "pos_y": 67.15625,
+ "pos_z": -94.9375,
+ "stations": "Fadlan City"
+ },
+ {
+ "name": "Rainbinan",
+ "pos_x": -102.15625,
+ "pos_y": -7.03125,
+ "pos_z": 92.625,
+ "stations": "Malaspina Dock,Merchiston Enterprise,Hambly Keep,Malocello Vision,Semeonis Settlement"
+ },
+ {
+ "name": "Rajgoney",
+ "pos_x": -68.59375,
+ "pos_y": 19.09375,
+ "pos_z": -117.375,
+ "stations": "Duffy Refinery,Bolotov Camp"
+ },
+ {
+ "name": "Rajgongga",
+ "pos_x": 25.90625,
+ "pos_y": -66.6875,
+ "pos_z": -133.9375,
+ "stations": "Fancher Port,Cayley Lab,Wallace Port"
+ },
+ {
+ "name": "Rajgonii",
+ "pos_x": 121.125,
+ "pos_y": -184,
+ "pos_z": 97.75,
+ "stations": "Libeskind Dock,Syromyatnikov Port,Opik Penal colony,Mead Terminal,Potocnik Town"
+ },
+ {
+ "name": "Rajgonta",
+ "pos_x": -54.9375,
+ "pos_y": -13.1875,
+ "pos_z": 90.875,
+ "stations": "Litke Station,Converse Ring,Nikitin Escape,Morin Terminal,Markham Base,Besonders Bastion"
+ },
+ {
+ "name": "Rajie",
+ "pos_x": -34.96875,
+ "pos_y": 108.03125,
+ "pos_z": 26.65625,
+ "stations": "Penn's Inheritance,Stanley Platform,Kettle Platform"
+ },
+ {
+ "name": "Rajita",
+ "pos_x": 80.0625,
+ "pos_y": 19.65625,
+ "pos_z": 98.9375,
+ "stations": "Agnesi Platform,Bulgarin Camp"
+ },
+ {
+ "name": "Rajito",
+ "pos_x": 125.9375,
+ "pos_y": -109.96875,
+ "pos_z": 21.28125,
+ "stations": "Sheremetevsky Terminal,Bullialdus Hub,Reeves Orbital,Koolhaas Orbital,Wickramasinghe Hub,Converse Vision,Goldberg Depot,Sandage Port,Hartog Holdings,Brosnan Enterprise,Orban Dock,Smyth Orbital,Baird Plant"
+ },
+ {
+ "name": "Rajuar",
+ "pos_x": -129.625,
+ "pos_y": -6.625,
+ "pos_z": -7.625,
+ "stations": "Donaldson Retreat"
+ },
+ {
+ "name": "Rajuarpai",
+ "pos_x": 132.125,
+ "pos_y": -162.5625,
+ "pos_z": -91.125,
+ "stations": "Mukai Reach"
+ },
+ {
+ "name": "Rajukru",
+ "pos_x": -96.9375,
+ "pos_y": 143.4375,
+ "pos_z": 4.625,
+ "stations": "Snyder Terminal,Van Scyoc Plant,Kerwin Station,Svavarsson Plant"
+ },
+ {
+ "name": "Rakapila",
+ "pos_x": -14.90625,
+ "pos_y": 33.625,
+ "pos_z": 9.125,
+ "stations": "Stone Enterprise,Walker Settlement"
+ },
+ {
+ "name": "Rakka",
+ "pos_x": -19.15625,
+ "pos_y": 164.28125,
+ "pos_z": -18.6875,
+ "stations": "Duke Survey,Baird Dock,Piercy Lab"
+ },
+ {
+ "name": "Rakkaia",
+ "pos_x": 13.8125,
+ "pos_y": -123.84375,
+ "pos_z": -19.78125,
+ "stations": "Inoda Settlement"
+ },
+ {
+ "name": "Rakkaiadi",
+ "pos_x": -52.6875,
+ "pos_y": -72.65625,
+ "pos_z": -7.15625,
+ "stations": "Shull Base,Bessemer Dock,Borisenko Enterprise,Fontenay Installation"
+ },
+ {
+ "name": "Rakkip",
+ "pos_x": 43.9375,
+ "pos_y": -114.03125,
+ "pos_z": -117.375,
+ "stations": "Tayler Beacon,Cook Asylum"
+ },
+ {
+ "name": "Raksaka",
+ "pos_x": 66.21875,
+ "pos_y": 16.34375,
+ "pos_z": 62.4375,
+ "stations": "Trevithick Port,Fettman Hub"
+ },
+ {
+ "name": "Raksha",
+ "pos_x": -36.46875,
+ "pos_y": -90.375,
+ "pos_z": -29.21875,
+ "stations": "McCandless Station,Garneau Hub,Sarafanov City,Whitney Orbital,Boswell Station,Camarda Port"
+ },
+ {
+ "name": "Rakshasas",
+ "pos_x": -80.625,
+ "pos_y": 87.03125,
+ "pos_z": 79.8125,
+ "stations": "Tognini Settlement,Markham Hub,Low Colony"
+ },
+ {
+ "name": "Raltekh",
+ "pos_x": -15.25,
+ "pos_y": 116.75,
+ "pos_z": -0.65625,
+ "stations": "Cavendish Survey,Vinge Platform,Bryant Settlement"
+ },
+ {
+ "name": "Ralteki",
+ "pos_x": -132.21875,
+ "pos_y": -83.5,
+ "pos_z": -16.125,
+ "stations": "Shaw Prospect"
+ },
+ {
+ "name": "Ralu",
+ "pos_x": 46.71875,
+ "pos_y": 1.4375,
+ "pos_z": -16.15625,
+ "stations": "Hartlib Plant,Viehbock Plant"
+ },
+ {
+ "name": "Ralunkas",
+ "pos_x": 181.375,
+ "pos_y": -159.03125,
+ "pos_z": 23.5,
+ "stations": "Lockyer Mines"
+ },
+ {
+ "name": "Raluvii",
+ "pos_x": -58.875,
+ "pos_y": -104.34375,
+ "pos_z": 67.65625,
+ "stations": "Fancher Market,Selous Dock"
+ },
+ {
+ "name": "Ramadillan",
+ "pos_x": 146.40625,
+ "pos_y": -43.375,
+ "pos_z": -17.4375,
+ "stations": "Lane Settlement,Baker Relay"
+ },
+ {
+ "name": "Raman",
+ "pos_x": 99.5625,
+ "pos_y": -138.375,
+ "pos_z": 55.34375,
+ "stations": "Reichelt Station"
+ },
+ {
+ "name": "Ramanco",
+ "pos_x": 19.71875,
+ "pos_y": -41.5,
+ "pos_z": 148.3125,
+ "stations": "Smith Base,Harrison Escape,Galiano Keep"
+ },
+ {
+ "name": "Ramandji",
+ "pos_x": 163.21875,
+ "pos_y": -58.5,
+ "pos_z": -32.59375,
+ "stations": "Wetherill Horizons,Busemann Settlement,Gurshtein Settlement,Nagata Terminal"
+ },
+ {
+ "name": "Ramapa",
+ "pos_x": 66.375,
+ "pos_y": -221.875,
+ "pos_z": 9.90625,
+ "stations": "Banneker Works,Suntzeff Base"
+ },
+ {
+ "name": "Ramapani",
+ "pos_x": 107.53125,
+ "pos_y": 27.75,
+ "pos_z": -105.6875,
+ "stations": "Crown Port"
+ },
+ {
+ "name": "Ramarga",
+ "pos_x": 60.53125,
+ "pos_y": 129.625,
+ "pos_z": 4.71875,
+ "stations": "Erdos Reformatory"
+ },
+ {
+ "name": "Ramine",
+ "pos_x": -57.5625,
+ "pos_y": 119.875,
+ "pos_z": 62.65625,
+ "stations": "Shepherd Dock,Daniel Platform,Regiomontanus Beacon,Belyayev Landing"
+ },
+ {
+ "name": "Ramit",
+ "pos_x": -91.0625,
+ "pos_y": -6.5,
+ "pos_z": 92.90625,
+ "stations": "Diesel Ring,Bolotov Dock,Khayyam City,Fernandes de Queiros Refinery"
+ },
+ {
+ "name": "Ramito",
+ "pos_x": 69.40625,
+ "pos_y": 117.9375,
+ "pos_z": 76.46875,
+ "stations": "Arnold Hub,Fraas Enterprise,Gamow Settlement,Yolen Installation,Benz Reach"
+ },
+ {
+ "name": "Rana",
+ "pos_x": 6.5,
+ "pos_y": -21,
+ "pos_z": -19.65625,
+ "stations": "Zholobov Gateway,Mullane Hub,Ali Hub,Edwards Enterprise,Wescott Hub"
+ },
+ {
+ "name": "Randgnid",
+ "pos_x": -9547.125,
+ "pos_y": -916.5,
+ "pos_z": 19832.5,
+ "stations": "Templar Barracks,Colonia Barracks"
+ },
+ {
+ "name": "Rang Gongs",
+ "pos_x": 111.71875,
+ "pos_y": 88.65625,
+ "pos_z": -95.4375,
+ "stations": "Hopkins Ring,Dampier Relay,Watson Vision,Artin Survey"
+ },
+ {
+ "name": "Rang Guans",
+ "pos_x": 106.4375,
+ "pos_y": 18.6875,
+ "pos_z": 11.84375,
+ "stations": "Acaba Laboratory,Hambly Works,Moore Terminal"
+ },
+ {
+ "name": "Rang Wang",
+ "pos_x": 43.53125,
+ "pos_y": 124.3125,
+ "pos_z": -111.84375,
+ "stations": "Macedo Sanctuary,Ziemkiewicz Vision"
+ },
+ {
+ "name": "Rangchan",
+ "pos_x": 58.3125,
+ "pos_y": 77.4375,
+ "pos_z": 127.21875,
+ "stations": "Bradbury Station,Boole Ring,Tilman Base,Sinisalo Relay"
+ },
+ {
+ "name": "Rangda",
+ "pos_x": 94.25,
+ "pos_y": -241.0625,
+ "pos_z": -24.03125,
+ "stations": "Abell Port,Milne's Claim"
+ },
+ {
+ "name": "Rangtei",
+ "pos_x": 88.71875,
+ "pos_y": -76.875,
+ "pos_z": 59.75,
+ "stations": "Lindblad Ring,Saarinen Station,Riazuddin Orbital,Schommer Port,Gentil City,Klein Town,Oterma Dock,Anderson Lab,Muller Ring,Nagata City,Whipple Terminal,Watts Settlement,Biggle Forum"
+ },
+ {
+ "name": "Rangur",
+ "pos_x": 86.78125,
+ "pos_y": -179.34375,
+ "pos_z": -24.5625,
+ "stations": "Xuesen Dock,Cartier Dock,Homer Barracks"
+ },
+ {
+ "name": "Raniyan",
+ "pos_x": 109.90625,
+ "pos_y": -35.375,
+ "pos_z": 118.875,
+ "stations": "Copernicus Dock,Meyrink Penal colony,Needham Platform"
+ },
+ {
+ "name": "Rapa Bao",
+ "pos_x": 94.15625,
+ "pos_y": -167.375,
+ "pos_z": -23.1875,
+ "stations": "Zhu Port,Flagg Gateway,Barcelona Terminal,Evans Vision,Wheeler Station,Skjellerup Station,Thomson Orbital,Knorre Reach,Chadwick's Progress,Haarsma Enterprise"
+ },
+ {
+ "name": "Rasa",
+ "pos_x": -81.5625,
+ "pos_y": 24.5,
+ "pos_z": 133.09375,
+ "stations": "Remec Dock"
+ },
+ {
+ "name": "Rasalhague",
+ "pos_x": -25.53125,
+ "pos_y": 17.5,
+ "pos_z": 34.9375,
+ "stations": "Vasyutin Dock,Bering City,Galvani Station,Kidman Terminal"
+ },
+ {
+ "name": "Rasani",
+ "pos_x": 136.03125,
+ "pos_y": -118.03125,
+ "pos_z": 130.84375,
+ "stations": "Wright Hub,Hind Mine"
+ },
+ {
+ "name": "Rasaste",
+ "pos_x": -27.53125,
+ "pos_y": -118.875,
+ "pos_z": -81.53125,
+ "stations": "Cormack Port,Haldeman Enterprise,Arber Dock,Lazarev Dock"
+ },
+ {
+ "name": "Rasmussen",
+ "pos_x": 17.34375,
+ "pos_y": -10.75,
+ "pos_z": -16.3125,
+ "stations": "Fort Forgie Starport,Fairbairn Settlement,Poincare Landing"
+ },
+ {
+ "name": "Rata Bares",
+ "pos_x": 72.0625,
+ "pos_y": -94.5625,
+ "pos_z": 36.09375,
+ "stations": "Anderson Refinery,Farghani's Folly"
+ },
+ {
+ "name": "Ratatosk",
+ "pos_x": -46.5625,
+ "pos_y": 68.875,
+ "pos_z": 84.5625,
+ "stations": "Lee Survey,Shirley Bastion"
+ },
+ {
+ "name": "Rataxo",
+ "pos_x": -14.34375,
+ "pos_y": -87.125,
+ "pos_z": 59.09375,
+ "stations": "Bus Hub,Narita Keep,Asire Stop"
+ },
+ {
+ "name": "Ratemere",
+ "pos_x": 45.53125,
+ "pos_y": -19.375,
+ "pos_z": 75.25,
+ "stations": "Forward Gateway,Ross Orbital,Tudela Dock,Rolland Port,Lee Ring,Ride Vision,Antonio de Andrade Enterprise,Barnaby Hub,Mayer Works,Polya Enterprise,Back Orbital,Burgess Point,Szentmartony Keep,Vess Prospect"
+ },
+ {
+ "name": "Rathakako",
+ "pos_x": -57.875,
+ "pos_y": -55.84375,
+ "pos_z": 38.375,
+ "stations": "Broglie Prospect,Brackett's Pride,Fast Outpost"
+ },
+ {
+ "name": "Rathamas",
+ "pos_x": 46.46875,
+ "pos_y": -153.78125,
+ "pos_z": 50.03125,
+ "stations": "Artzybasheff Hub,Lerner Terminal"
+ },
+ {
+ "name": "Rathawa",
+ "pos_x": -82.125,
+ "pos_y": -103.15625,
+ "pos_z": 56.09375,
+ "stations": "Stephenson Mine,Fettman Works"
+ },
+ {
+ "name": "Rati Irtii",
+ "pos_x": 37.9375,
+ "pos_y": -33.90625,
+ "pos_z": 85.1875,
+ "stations": "Newcomen City,Mayer Enterprise,McMonagle Hub,Bohr Prospect,Fox Depot"
+ },
+ {
+ "name": "Ratici",
+ "pos_x": 3.34375,
+ "pos_y": -238.875,
+ "pos_z": 23.1875,
+ "stations": "Ziemkiewicz Installation,Mainzer Mines,Bassford Station"
+ },
+ {
+ "name": "Ratis",
+ "pos_x": -122.40625,
+ "pos_y": 30.15625,
+ "pos_z": 75.0625,
+ "stations": "Fincke Orbital,Selye Point,Teng-hui Orbital"
+ },
+ {
+ "name": "Ratraii",
+ "pos_x": -9530.96875,
+ "pos_y": -918.03125,
+ "pos_z": 19803.8125,
+ "stations": "Colonia Dream,Bonestell Point,Exodus Reach"
+ },
+ {
+ "name": "Ratri",
+ "pos_x": -11.53125,
+ "pos_y": 70,
+ "pos_z": -26.75,
+ "stations": "Sterling Ring"
+ },
+ {
+ "name": "Raugeni",
+ "pos_x": 62.625,
+ "pos_y": -15.125,
+ "pos_z": -6.4375,
+ "stations": "Crouch Hub,Sherrington Terminal"
+ },
+ {
+ "name": "Raugenik",
+ "pos_x": -68,
+ "pos_y": -129.46875,
+ "pos_z": -20.21875,
+ "stations": "Charnas Orbital,Cherry Penal colony,Bickel Base"
+ },
+ {
+ "name": "Raugeth",
+ "pos_x": 15.25,
+ "pos_y": 17.375,
+ "pos_z": 128.4375,
+ "stations": "Bernoulli Landing"
+ },
+ {
+ "name": "Rauraci",
+ "pos_x": 102.59375,
+ "pos_y": -118.71875,
+ "pos_z": 52.125,
+ "stations": "Cyrrhus Survey,Watson Arsenal,Ferguson Mines,Connes Survey"
+ },
+ {
+ "name": "Raurakmo",
+ "pos_x": -49.3125,
+ "pos_y": -154.96875,
+ "pos_z": -31.71875,
+ "stations": "Inoda Dock,Hoffleit Horizons,Nijland Silo"
+ },
+ {
+ "name": "Rauras",
+ "pos_x": -32.0625,
+ "pos_y": 92.75,
+ "pos_z": -64.53125,
+ "stations": "Williams Terminal,Krupkat Orbital,Makarov City,Taylor Enterprise,Smith City,Ricci City,Cole Port,Leiber Settlement"
+ },
+ {
+ "name": "Rauratunab",
+ "pos_x": -118.4375,
+ "pos_y": 11.03125,
+ "pos_z": -51.78125,
+ "stations": "Plante Prospect"
+ },
+ {
+ "name": "Raurici",
+ "pos_x": 81.40625,
+ "pos_y": -128.53125,
+ "pos_z": 0.75,
+ "stations": "Armero Platform,Flammarion Mines"
+ },
+ {
+ "name": "Raurt",
+ "pos_x": 148.25,
+ "pos_y": 56.46875,
+ "pos_z": -37.4375,
+ "stations": "Davis Mines,Holub Port,Ellison Enterprise,Normand Point"
+ },
+ {
+ "name": "Raurubo",
+ "pos_x": -4.8125,
+ "pos_y": -178.6875,
+ "pos_z": 5.96875,
+ "stations": "Pierce Settlement,Verrier Port,Wilhelm von Struve Port"
+ },
+ {
+ "name": "Raurugba",
+ "pos_x": 81.21875,
+ "pos_y": -46.21875,
+ "pos_z": 22.40625,
+ "stations": "Jackson Hub,Endate Enterprise,Ritchey Landing"
+ },
+ {
+ "name": "Raurumbal",
+ "pos_x": 59.8125,
+ "pos_y": -84.5,
+ "pos_z": -40.9375,
+ "stations": "Dickinson Vision,Youll Enterprise,Palmer Bastion"
+ },
+ {
+ "name": "Raurus",
+ "pos_x": 97.40625,
+ "pos_y": -42.15625,
+ "pos_z": 75.03125,
+ "stations": "Watson Beacon"
+ },
+ {
+ "name": "Rauta",
+ "pos_x": 31.21875,
+ "pos_y": -69.09375,
+ "pos_z": 86.46875,
+ "stations": "Giraud Station,Herzog Hub,Vogel Keep"
+ },
+ {
+ "name": "Rautama",
+ "pos_x": -124.46875,
+ "pos_y": -13.09375,
+ "pos_z": -10.5,
+ "stations": "Melroy Market,Sarich Horizons,Porges Penal colony"
+ },
+ {
+ "name": "Rautandji",
+ "pos_x": 106.90625,
+ "pos_y": -95.375,
+ "pos_z": 121.8125,
+ "stations": "Tarter City,Naylor Port,Boyajian Vision,Tshang Landing,McKee Prospect"
+ },
+ {
+ "name": "Ravanahutis",
+ "pos_x": -77.09375,
+ "pos_y": 19.5625,
+ "pos_z": 35.4375,
+ "stations": "Lounge Terminal"
+ },
+ {
+ "name": "Ravane",
+ "pos_x": 42.46875,
+ "pos_y": 61.71875,
+ "pos_z": -137.15625,
+ "stations": "Plexico Hub,Ahern Platform"
+ },
+ {
+ "name": "Ravas",
+ "pos_x": 32.125,
+ "pos_y": 24.21875,
+ "pos_z": 92.8125,
+ "stations": "Issigonis Plant,Vian Horizons,Kinsey Station"
+ },
+ {
+ "name": "Ravenhold",
+ "pos_x": -9548.46875,
+ "pos_y": -938.625,
+ "pos_z": 19801.8125,
+ "stations": "Karrison's Landing"
+ },
+ {
+ "name": "RBS 1843",
+ "pos_x": -17.1875,
+ "pos_y": -25.21875,
+ "pos_z": 13.25,
+ "stations": "Bernard Landing,Scott Orbital,Nyberg Dock,Piercy Terminal"
+ },
+ {
+ "name": "RBS 742",
+ "pos_x": 7,
+ "pos_y": -34.09375,
+ "pos_z": -39.46875,
+ "stations": "Hovell Arsenal,Clayton Lab,Markham Installation,Neumann Relay"
+ },
+ {
+ "name": "Recua",
+ "pos_x": 51.9375,
+ "pos_y": -77.25,
+ "pos_z": 21.3125,
+ "stations": "Gorey Enterprise,Baillaud Base,Meuron Terminal"
+ },
+ {
+ "name": "Recuanque",
+ "pos_x": 63.65625,
+ "pos_y": 23.21875,
+ "pos_z": -102.1875,
+ "stations": "Fuglesang City,Peano Penal colony,Vishweswarayya Enterprise,Jendrassik's Pride,Akiyama Dock,Pryor Observatory"
+ },
+ {
+ "name": "Recuayala",
+ "pos_x": 92.09375,
+ "pos_y": -58.875,
+ "pos_z": -69.84375,
+ "stations": "Somerset Dock,McClintock Terminal,Smith's Inheritance"
+ },
+ {
+ "name": "Reddi Dhod",
+ "pos_x": 40.4375,
+ "pos_y": -101.96875,
+ "pos_z": 140.6875,
+ "stations": "Meech Platform,Helffrich Vision,Goonan Refinery,Piercy Works"
+ },
+ {
+ "name": "Reddis",
+ "pos_x": 137.46875,
+ "pos_y": -169.25,
+ "pos_z": 67.59375,
+ "stations": "Ahnert-Rohlfs Gateway,Tyson Plant,Olahus Port,Chadbourne Station,Mozhaysky Bastion"
+ },
+ {
+ "name": "Reddot",
+ "pos_x": 16.03125,
+ "pos_y": -75.3125,
+ "pos_z": 10.0625,
+ "stations": "Whit's Station"
+ },
+ {
+ "name": "Redoneangi",
+ "pos_x": 131.21875,
+ "pos_y": -90.8125,
+ "pos_z": 39.4375,
+ "stations": "Schmidt Vision"
+ },
+ {
+ "name": "Redonesses",
+ "pos_x": -55.4375,
+ "pos_y": 148.8125,
+ "pos_z": -87.34375,
+ "stations": "Ellern Orbital,Monge Enterprise,Shapiro Colony,Borel Terminal,Cartier Keep"
+ },
+ {
+ "name": "Redong Goab",
+ "pos_x": 44.78125,
+ "pos_y": 44.4375,
+ "pos_z": 90.5625,
+ "stations": "Meyrink Orbital"
+ },
+ {
+ "name": "Regi",
+ "pos_x": -129.4375,
+ "pos_y": 47.3125,
+ "pos_z": -13.625,
+ "stations": "Jeury Port,Rasmussen Dock,Leiber Orbital"
+ },
+ {
+ "name": "Regina",
+ "pos_x": 55.53125,
+ "pos_y": 150.0625,
+ "pos_z": 81.28125,
+ "stations": "McDivitt Platform,Kregel Camp"
+ },
+ {
+ "name": "Regir",
+ "pos_x": 86.21875,
+ "pos_y": -140.34375,
+ "pos_z": 20.53125,
+ "stations": "Ikeya Terminal,Grushin Dock,Suzuki Orbital,Davidson Penal colony"
+ },
+ {
+ "name": "Regira",
+ "pos_x": 20.46875,
+ "pos_y": -54.34375,
+ "pos_z": 71.75,
+ "stations": "Clayton Orbital,Bordage Port,Samuda Dock,Bunch Orbital,Lee Port,Furukawa Vista,Lorrah Penal colony,Beekman Lab,Citi Terminal,Glenn's Folly,Anders Holdings,Garneau Prospect"
+ },
+ {
+ "name": "Regirina",
+ "pos_x": -56.90625,
+ "pos_y": 39.9375,
+ "pos_z": 119.0625,
+ "stations": "Tesla Orbital"
+ },
+ {
+ "name": "Regnenses",
+ "pos_x": 114.03125,
+ "pos_y": -2.4375,
+ "pos_z": 10.3125,
+ "stations": "Haller Orbital,Chiao Station,Gemar Port,Irens Bastion,Vinge Camp"
+ },
+ {
+ "name": "Regulus",
+ "pos_x": 37.875,
+ "pos_y": 60.1875,
+ "pos_z": -35.0625,
+ "stations": "Papin Orbital"
+ },
+ {
+ "name": "Reien Di",
+ "pos_x": 132.90625,
+ "pos_y": -128.46875,
+ "pos_z": 111.25,
+ "stations": "Dornier Port"
+ },
+ {
+ "name": "Reienadi",
+ "pos_x": 88,
+ "pos_y": -174.8125,
+ "pos_z": 151.1875,
+ "stations": "Kosai Mines,Korolyov Landing,Moffat Enterprise,Babcock Silo"
+ },
+ {
+ "name": "Reienete",
+ "pos_x": 128.09375,
+ "pos_y": 2.6875,
+ "pos_z": -88.25,
+ "stations": "Czerneda Enterprise,Pippin Relay,Eisele Orbital"
+ },
+ {
+ "name": "Reieni",
+ "pos_x": 147.1875,
+ "pos_y": -9,
+ "pos_z": 46.78125,
+ "stations": "Sellings Penal colony,Land Outpost,Zebrowski Observatory"
+ },
+ {
+ "name": "Reiens",
+ "pos_x": 142.09375,
+ "pos_y": -147.875,
+ "pos_z": 64.90625,
+ "stations": "Pacheco Base,Fokker Mines"
+ },
+ {
+ "name": "Reiensei",
+ "pos_x": 23.65625,
+ "pos_y": -157.09375,
+ "pos_z": -75.6875,
+ "stations": "Kamov Orbital,Plait Orbital"
+ },
+ {
+ "name": "Reienti",
+ "pos_x": -57.90625,
+ "pos_y": -102.90625,
+ "pos_z": 77.3125,
+ "stations": "Mikulin Ring,McKie Colony,Paulmier de Gonneville Survey,Gardner Survey"
+ },
+ {
+ "name": "Remil",
+ "pos_x": 80.59375,
+ "pos_y": 97.5,
+ "pos_z": 60.71875,
+ "stations": "Schwann Holdings,Tryggvason Camp"
+ },
+ {
+ "name": "Remines",
+ "pos_x": -28.65625,
+ "pos_y": -23.21875,
+ "pos_z": -71.40625,
+ "stations": "Crick Terminal,Bobko Hub,Blenkinsop Hub,Resnik Dock,Nye Settlement,Porsche Gateway,Matthews Works,Viehbock Dock,Behring Ring,Sinisalo Works,McCandless Settlement,Guest Hub"
+ },
+ {
+ "name": "Ren Tun",
+ "pos_x": 78.1875,
+ "pos_y": -162.5625,
+ "pos_z": -30.40625,
+ "stations": "Houten-Groeneveld Mine,MacLeod Depot"
+ },
+ {
+ "name": "Renef",
+ "pos_x": -10.40625,
+ "pos_y": -200.8125,
+ "pos_z": 75.59375,
+ "stations": "Syromyatnikov Beacon,Wood Relay"
+ },
+ {
+ "name": "Reneru",
+ "pos_x": -111.9375,
+ "pos_y": -24.0625,
+ "pos_z": 106.71875,
+ "stations": "Grunsfeld Outpost"
+ },
+ {
+ "name": "Renet",
+ "pos_x": 115.46875,
+ "pos_y": -9.5625,
+ "pos_z": 75.6875,
+ "stations": "Anthony de la Roche Dock,Shaver Enterprise,Chiang Vision,Alexander Base"
+ },
+ {
+ "name": "Renetes",
+ "pos_x": -14.09375,
+ "pos_y": -8.875,
+ "pos_z": -88.5625,
+ "stations": "Ali Refinery,Ansari Point,Stanier City"
+ },
+ {
+ "name": "Renpa",
+ "pos_x": 191.3125,
+ "pos_y": -124.71875,
+ "pos_z": 35.21875,
+ "stations": "Elbakyan Survey"
+ },
+ {
+ "name": "Renpeians",
+ "pos_x": 80.4375,
+ "pos_y": -95.40625,
+ "pos_z": 164.90625,
+ "stations": "Osterbrock Terminal,Abetti Installation"
+ },
+ {
+ "name": "Renpetani",
+ "pos_x": -110.0625,
+ "pos_y": -50.1875,
+ "pos_z": -89.0625,
+ "stations": "Henize Survey"
+ },
+ {
+ "name": "Reorte",
+ "pos_x": 75.75,
+ "pos_y": 48.75,
+ "pos_z": 75.15625,
+ "stations": "Davies High,Garcia Horizons,Almagro Port,Baird Bastion,Alenquer Enterprise"
+ },
+ {
+ "name": "Rerebatz",
+ "pos_x": 66.71875,
+ "pos_y": -208.4375,
+ "pos_z": 107.03125,
+ "stations": "Marsden Point"
+ },
+ {
+ "name": "Reret",
+ "pos_x": 32.75,
+ "pos_y": -164.9375,
+ "pos_z": -2.3125,
+ "stations": "Grunsfeld Gateway,Kreutz Dock,Sewell Vision,Hoffman Prospect,Ogden Legacy"
+ },
+ {
+ "name": "Reshas",
+ "pos_x": 73.9375,
+ "pos_y": 71.03125,
+ "pos_z": 54.59375,
+ "stations": "Banks Port,Luiken City"
+ },
+ {
+ "name": "Reshero",
+ "pos_x": -2.59375,
+ "pos_y": -162.84375,
+ "pos_z": -8.90625,
+ "stations": "Lambert Ring,Bharadwaj Silo"
+ },
+ {
+ "name": "Reshesh",
+ "pos_x": 193.65625,
+ "pos_y": -135.84375,
+ "pos_z": 33.8125,
+ "stations": "Humason Terminal,Alenquer Vision,Impey Port,Burns Port,Gibson Enterprise"
+ },
+ {
+ "name": "Reynes",
+ "pos_x": 82.21875,
+ "pos_y": -131.78125,
+ "pos_z": -0.84375,
+ "stations": "Embakasi,Fallows Base,Coblentz Camp"
+ },
+ {
+ "name": "Rhea",
+ "pos_x": 58.125,
+ "pos_y": 22.59375,
+ "pos_z": -28.59375,
+ "stations": "Ito Orbital,Carter Port,Foreman Holdings,Balandin Gateway,Broglie Ring,Cormack Penal colony,de Sousa Terminal"
+ },
+ {
+ "name": "Rhiannon",
+ "pos_x": -22.90625,
+ "pos_y": -42.09375,
+ "pos_z": -8.84375,
+ "stations": "Dyson Hub"
+ },
+ {
+ "name": "Rhibo",
+ "pos_x": 80.40625,
+ "pos_y": -136.78125,
+ "pos_z": 17.3125,
+ "stations": "Oort Refinery,Keldysh Enterprise,Bullialdus Settlement"
+ },
+ {
+ "name": "Rhibonii",
+ "pos_x": -72.90625,
+ "pos_y": -50.5,
+ "pos_z": -91.96875,
+ "stations": "Komarov Base"
+ },
+ {
+ "name": "Rho Andromedae",
+ "pos_x": -128.625,
+ "pos_y": -65.1875,
+ "pos_z": -65.46875,
+ "stations": "Anderson Point"
+ },
+ {
+ "name": "Rho Cancri",
+ "pos_x": 9.34375,
+ "pos_y": 24.9375,
+ "pos_z": -30.1875,
+ "stations": "Dekker Base,Hamilton Reserve,Cayley Orbital,Andrews Colony,Lefschetz Gateway,Liwei Keep"
+ },
+ {
+ "name": "Rho Capricorni",
+ "pos_x": -39.25,
+ "pos_y": -49.125,
+ "pos_z": 76.09375,
+ "stations": "Loncke Prospect,Rutan Plant,Pond Landing"
+ },
+ {
+ "name": "Rho Geminorum",
+ "pos_x": 7.09375,
+ "pos_y": 22,
+ "pos_z": -54.15625,
+ "stations": "Macquorn Rankine Gateway,Khan City,Whitworth Station,Drew Gateway,Wang Ring,Crick Enterprise,Good Ring"
+ },
+ {
+ "name": "Rho Gruis",
+ "pos_x": 7.53125,
+ "pos_y": -201.0625,
+ "pos_z": 112.125,
+ "stations": "Abetti Camp,Wales Depot"
+ },
+ {
+ "name": "Rho Indi",
+ "pos_x": 43.21875,
+ "pos_y": -60.9375,
+ "pos_z": 45.03125,
+ "stations": "Erikson Retreat"
+ },
+ {
+ "name": "Rho Octantis",
+ "pos_x": 157.875,
+ "pos_y": -85.4375,
+ "pos_z": 119.59375,
+ "stations": "Melotte Relay"
+ },
+ {
+ "name": "Rho Pavonis",
+ "pos_x": 64.375,
+ "pos_y": -113.34375,
+ "pos_z": 137.71875,
+ "stations": "Hay Settlement,Tanaka Settlement,Warner Hub"
+ },
+ {
+ "name": "Rho Phoenicis",
+ "pos_x": 81.84375,
+ "pos_y": -221.9375,
+ "pos_z": 51.8125,
+ "stations": "Gould Terminal,Hodgson Point,Oberth Landing,Rangarajan Settlement"
+ },
+ {
+ "name": "Rho Puppis",
+ "pos_x": 56.6875,
+ "pos_y": 5.21875,
+ "pos_z": -28.25,
+ "stations": "Song Orbital"
+ },
+ {
+ "name": "Rho Telescopii",
+ "pos_x": 44.34375,
+ "pos_y": -75,
+ "pos_z": 163.1875,
+ "stations": "Farmer Platform"
+ },
+ {
+ "name": "Rho Tucanae",
+ "pos_x": 68.34375,
+ "pos_y": -105.375,
+ "pos_z": 46.25,
+ "stations": "Lewis Survey,Michell Prospect"
+ },
+ {
+ "name": "Rian",
+ "pos_x": 74.5,
+ "pos_y": -216.40625,
+ "pos_z": 55.8125,
+ "stations": "Airy Reformatory"
+ },
+ {
+ "name": "Rianik",
+ "pos_x": -75.25,
+ "pos_y": -85.0625,
+ "pos_z": 139.875,
+ "stations": "Kondakova's Progress,Gregory Settlement,Leichhardt's Inheritance"
+ },
+ {
+ "name": "Rianindana",
+ "pos_x": 64.96875,
+ "pos_y": -156.96875,
+ "pos_z": 108.5625,
+ "stations": "Giacobini Port,Sheremetevsky Holdings,Barnard Dock,Webb Penal colony"
+ },
+ {
+ "name": "Rians",
+ "pos_x": 95.28125,
+ "pos_y": 58.71875,
+ "pos_z": 99.125,
+ "stations": "Morris Orbital,Dedman Mines,Fraser Port,Shunn Vision"
+ },
+ {
+ "name": "Ribhniugo",
+ "pos_x": 18.5,
+ "pos_y": 82.3125,
+ "pos_z": -13.25,
+ "stations": "Pasteur Refinery,Afanasyev Landing,Chilton Colony"
+ },
+ {
+ "name": "Riedquat",
+ "pos_x": 68.84375,
+ "pos_y": 48.75,
+ "pos_z": 69.75,
+ "stations": "La Soeur du Dan Ham,Lavoisier Dock,Marshall Dock"
+ },
+ {
+ "name": "Rig Vegas",
+ "pos_x": 104.03125,
+ "pos_y": 42.15625,
+ "pos_z": -17.78125,
+ "stations": "Sargent Horizons,Linaweaver Installation"
+ },
+ {
+ "name": "Rig Vegast",
+ "pos_x": -106.84375,
+ "pos_y": -32.75,
+ "pos_z": -21.53125,
+ "stations": "Sarich Landing,Scithers Bastion"
+ },
+ {
+ "name": "Rigo",
+ "pos_x": 59.09375,
+ "pos_y": 14.625,
+ "pos_z": -86.125,
+ "stations": "Timofeyevich Hub"
+ },
+ {
+ "name": "Rigoneskher",
+ "pos_x": -10.375,
+ "pos_y": -82.96875,
+ "pos_z": 15.8125,
+ "stations": "Perrine Landing,Lemonnier Survey"
+ },
+ {
+ "name": "Rigosage",
+ "pos_x": -44.09375,
+ "pos_y": 136.90625,
+ "pos_z": 77.96875,
+ "stations": "Moresby Orbital"
+ },
+ {
+ "name": "Rigosages",
+ "pos_x": 16.53125,
+ "pos_y": -6.15625,
+ "pos_z": -133.40625,
+ "stations": "Hutton Horizons,Wright Point,Zelazny Base"
+ },
+ {
+ "name": "Rikba Atius",
+ "pos_x": 40.6875,
+ "pos_y": -2.75,
+ "pos_z": 120.875,
+ "stations": "Webb Dock,Watts Plant,Isherwood Hub"
+ },
+ {
+ "name": "Rikbakar",
+ "pos_x": -129.90625,
+ "pos_y": 116.96875,
+ "pos_z": -0.90625,
+ "stations": "Frechet City,Bombelli Settlement,Gulyaev Arsenal"
+ },
+ {
+ "name": "Rikbakara",
+ "pos_x": 112.96875,
+ "pos_y": -63.25,
+ "pos_z": -122.78125,
+ "stations": "Peirce Sanctuary,Brosnan Works"
+ },
+ {
+ "name": "Rikbakshmi",
+ "pos_x": -29.75,
+ "pos_y": 164.875,
+ "pos_z": 18.5625,
+ "stations": "Mitchell Vision,Lefschetz Enterprise,Macedo Orbital,Kovalevskaya Works"
+ },
+ {
+ "name": "Riki",
+ "pos_x": 93,
+ "pos_y": -5.6875,
+ "pos_z": 19.71875,
+ "stations": "Bischoff Installation,Langford Prospect"
+ },
+ {
+ "name": "Rind",
+ "pos_x": 77.875,
+ "pos_y": -79.90625,
+ "pos_z": 35.9375,
+ "stations": "Beer Orbital,Dornier Prospect,Baade Orbital,Heinemann Orbital,Wegner Market,Cellarius Hub,Stone Dock,Ryan Port,Bigourdan Vista,Banks Vision,Burbidge Colony,Wallin Settlement,Horrocks Hub,Brunner Survey,Pickering Port"
+ },
+ {
+ "name": "Rindjalavs",
+ "pos_x": 100.40625,
+ "pos_y": -57.28125,
+ "pos_z": -32.0625,
+ "stations": "Battani Landing,Kresak Holdings"
+ },
+ {
+ "name": "Rindji",
+ "pos_x": 68.25,
+ "pos_y": -145.125,
+ "pos_z": -57.1875,
+ "stations": "Silverberg Horizons,Guin Hub,Scithers Enterprise"
+ },
+ {
+ "name": "Rindjibal",
+ "pos_x": 133,
+ "pos_y": -3.375,
+ "pos_z": 23.875,
+ "stations": "Lopez de Legazpi Orbital,Hartog Station,Rocklynne Barracks,Wul Port"
+ },
+ {
+ "name": "Rindjigas",
+ "pos_x": 148.9375,
+ "pos_y": -158.625,
+ "pos_z": -48.125,
+ "stations": "Gill Platform"
+ },
+ {
+ "name": "Rindrani",
+ "pos_x": 117.65625,
+ "pos_y": 38.0625,
+ "pos_z": -123.9375,
+ "stations": "Appel Mines"
+ },
+ {
+ "name": "Ring",
+ "pos_x": 33.4375,
+ "pos_y": -94.65625,
+ "pos_z": -130.71875,
+ "stations": "Gerlache Installation,Hippalus Sanctuary"
+ },
+ {
+ "name": "Ringardha",
+ "pos_x": -5.6875,
+ "pos_y": 73.78125,
+ "pos_z": -45.1875,
+ "stations": "Britnev Horizons,Lagrange Gateway,Teng Terminal,Frick Base,Sarmiento de Gamboa Hub"
+ },
+ {
+ "name": "Ringgati",
+ "pos_x": 70.8125,
+ "pos_y": -2.40625,
+ "pos_z": -131.03125,
+ "stations": "Ahmed Base,Naddoddur Outpost"
+ },
+ {
+ "name": "Rio Paha",
+ "pos_x": 71.375,
+ "pos_y": -145.90625,
+ "pos_z": 161.96875,
+ "stations": "Orban Base"
+ },
+ {
+ "name": "Rio Pahads",
+ "pos_x": 106.75,
+ "pos_y": 95.78125,
+ "pos_z": 92.1875,
+ "stations": "Bova City,Heaviside's Inheritance,Hennepin Terminal"
+ },
+ {
+ "name": "Rio Po",
+ "pos_x": 79.625,
+ "pos_y": -25.5625,
+ "pos_z": -64.25,
+ "stations": "Ride Enterprise,Bunsen Terminal,Laue Platform,Hire Landing,Reynolds Plant"
+ },
+ {
+ "name": "Rish",
+ "pos_x": -23.5,
+ "pos_y": 9.125,
+ "pos_z": 62.34375,
+ "stations": "Harbaugh Hub,Moore Works,Salk Orbital"
+ },
+ {
+ "name": "Rishadi",
+ "pos_x": 138.46875,
+ "pos_y": -27.0625,
+ "pos_z": 53.5,
+ "stations": "Poncelet Outpost"
+ },
+ {
+ "name": "Rishair",
+ "pos_x": 95.71875,
+ "pos_y": -160.46875,
+ "pos_z": 40.46875,
+ "stations": "Lindblad Port,Lindstrand Station,Pellegrino Point,Thomson Plant,Doctorow's Folly,Kreutz City"
+ },
+ {
+ "name": "Rishalu",
+ "pos_x": 18.625,
+ "pos_y": -32.25,
+ "pos_z": 59.4375,
+ "stations": "Pryor's Claim"
+ },
+ {
+ "name": "Rishanha",
+ "pos_x": 27.40625,
+ "pos_y": -179.25,
+ "pos_z": -23.65625,
+ "stations": "Karachkina Orbital,Stevin Hub,Brosnatch Ring"
+ },
+ {
+ "name": "Rishatkwali",
+ "pos_x": 72.625,
+ "pos_y": -141.3125,
+ "pos_z": 101.90625,
+ "stations": "Mouchez Gateway,Picacio Hub,Webb Orbital,Whelan Station,Gordon Point,Ortiz Moreno Silo,Darwin City,Mackenzie Point,Bainbridge Hub,Hill Point,Pollas Holdings,Francisco de Eliza Vision"
+ },
+ {
+ "name": "Rishia",
+ "pos_x": 155.375,
+ "pos_y": 69.71875,
+ "pos_z": -9.8125,
+ "stations": "Lerner Platform,Doyle Colony,Hannu Keep,Spielberg Prospect"
+ },
+ {
+ "name": "Rishishai",
+ "pos_x": 79.09375,
+ "pos_y": -82.09375,
+ "pos_z": 115.8125,
+ "stations": "Shen Platform,Elst Refinery,MacCready Vision"
+ },
+ {
+ "name": "Rishmi",
+ "pos_x": -1.40625,
+ "pos_y": -75.15625,
+ "pos_z": 107.71875,
+ "stations": "Elvstrom Point,Brooks' Progress"
+ },
+ {
+ "name": "Rishnum",
+ "pos_x": -140.25,
+ "pos_y": 20.84375,
+ "pos_z": -40.8125,
+ "stations": "Gooch Port,Pythagoras Depot"
+ },
+ {
+ "name": "Rishpakos",
+ "pos_x": 86.3125,
+ "pos_y": -27.625,
+ "pos_z": 58.59375,
+ "stations": "Simonyi Platform"
+ },
+ {
+ "name": "Rishtrigu",
+ "pos_x": 23.59375,
+ "pos_y": -145.21875,
+ "pos_z": 30.53125,
+ "stations": "Abell Terminal,de Kamp Refinery,Hansen Landing"
+ },
+ {
+ "name": "Ritila",
+ "pos_x": 86.59375,
+ "pos_y": 60.8125,
+ "pos_z": 71.28125,
+ "stations": "Kennedy Dock,Narbeth Installation,Younghusband Colony,Gardner Relay"
+ },
+ {
+ "name": "RMK 6",
+ "pos_x": 98.375,
+ "pos_y": -30.03125,
+ "pos_z": -10.8125,
+ "stations": "Zudov Hub,Blair Prospect,Clement Ring,Brongniart Park,Rescue Ship - Zudov Hub"
+ },
+ {
+ "name": "Robigo",
+ "pos_x": -303.40625,
+ "pos_y": 7.3125,
+ "pos_z": -314.15625,
+ "stations": "Robigo Mines,Hauser's Reach,Sirotanovic's Legacy,Quaid's Vision"
+ },
+ {
+ "name": "Robogassee",
+ "pos_x": 122.53125,
+ "pos_y": 28.84375,
+ "pos_z": 18.90625,
+ "stations": "Winne Enterprise,Haber Ring,Celsius Station,Fox Silo,Mitropoulos Depot"
+ },
+ {
+ "name": "Robolda",
+ "pos_x": 2,
+ "pos_y": -150.375,
+ "pos_z": 125.71875,
+ "stations": "Iben Vision,Giclas Terminal,Fadlan's Claim"
+ },
+ {
+ "name": "Roboldyanna",
+ "pos_x": 91.8125,
+ "pos_y": 39.15625,
+ "pos_z": 120.65625,
+ "stations": "Willis Hub,King Bastion"
+ },
+ {
+ "name": "Robole",
+ "pos_x": -100.5,
+ "pos_y": 62.96875,
+ "pos_z": 40.59375,
+ "stations": "Lamarck Terminal"
+ },
+ {
+ "name": "Robor",
+ "pos_x": 58.5,
+ "pos_y": 64.8125,
+ "pos_z": 30.3125,
+ "stations": "Hooke Vision,Bulychev Installation"
+ },
+ {
+ "name": "Rod",
+ "pos_x": -3.21875,
+ "pos_y": -50.5625,
+ "pos_z": 0.6875,
+ "stations": "Gohar Landing,Low City,Campbell Installation"
+ },
+ {
+ "name": "Rodentia",
+ "pos_x": -9530.53125,
+ "pos_y": -907.25,
+ "pos_z": 19787.375,
+ "stations": "Surly's Nest"
+ },
+ {
+ "name": "Rodentia Petram",
+ "pos_x": -9521.59375,
+ "pos_y": -896.5625,
+ "pos_z": 19844,
+ "stations": "Rock Rats Reach"
+ },
+ {
+ "name": "Rohini",
+ "pos_x": -3374.8125,
+ "pos_y": -47.8125,
+ "pos_z": 6912.25,
+ "stations": "Eudoxus Hithe,Eudaemon Anchorage"
+ },
+ {
+ "name": "Rong",
+ "pos_x": -18.375,
+ "pos_y": 13.125,
+ "pos_z": -97.75,
+ "stations": "Fettman Ring,Macomb Bastion,Kuhn City,Detmer Landing"
+ },
+ {
+ "name": "Ronggi",
+ "pos_x": 167.21875,
+ "pos_y": -117.28125,
+ "pos_z": 27.34375,
+ "stations": "Hariot Survey,Marius Beacon,Krylov Terminal"
+ },
+ {
+ "name": "Rongites",
+ "pos_x": 87.09375,
+ "pos_y": -61.8125,
+ "pos_z": -80.28125,
+ "stations": "Soto Camp,Bacigalupi Terminal,Tayler Vision"
+ },
+ {
+ "name": "Rongxians",
+ "pos_x": 121.5,
+ "pos_y": -105.75,
+ "pos_z": 45.8125,
+ "stations": "Tarter Port,Maitz Vision,Gunter Terminal,Varley Prospect,Conklin Point"
+ },
+ {
+ "name": "Rosette Sector CQ-Y d59",
+ "pos_x": 2345.96875,
+ "pos_y": -167.4375,
+ "pos_z": -4752.90625,
+ "stations": "New Beginning"
+ },
+ {
+ "name": "Rosmerta",
+ "pos_x": 65.375,
+ "pos_y": -157.125,
+ "pos_z": 42.34375,
+ "stations": "Kahn Orbital,Samos Terminal,Dugan Vision,Reid's Progress,Rabinowitz's Folly"
+ },
+ {
+ "name": "Ross 1003",
+ "pos_x": -4.3125,
+ "pos_y": 33.90625,
+ "pos_z": -11.96875,
+ "stations": "Milnor Penal colony,Zindell Platform"
+ },
+ {
+ "name": "Ross 1008",
+ "pos_x": -8.71875,
+ "pos_y": 51.5625,
+ "pos_z": 1.5,
+ "stations": "Yamazaki Hub"
+ },
+ {
+ "name": "Ross 1015",
+ "pos_x": -6.09375,
+ "pos_y": 29.46875,
+ "pos_z": 3.03125,
+ "stations": "Bowersox Mines,Hand Hub"
+ },
+ {
+ "name": "Ross 1028b",
+ "pos_x": -6.78125,
+ "pos_y": 66,
+ "pos_z": 44.65625,
+ "stations": "Glashow Colony"
+ },
+ {
+ "name": "Ross 104",
+ "pos_x": 5.5625,
+ "pos_y": 19.6875,
+ "pos_z": -7.3125,
+ "stations": "Bunch City,Shiner Port,Penn Ring,Creighton Landing,Allen Silo"
+ },
+ {
+ "name": "Ross 1047",
+ "pos_x": -1.84375,
+ "pos_y": 35.34375,
+ "pos_z": 31.09375,
+ "stations": "Drew Dock,Mastracchio Orbital,Cortes Base,Humboldt Lab"
+ },
+ {
+ "name": "Ross 1051",
+ "pos_x": -37.21875,
+ "pos_y": 44.5,
+ "pos_z": -5.0625,
+ "stations": "Abetti Platform,Galois Legacy"
+ },
+ {
+ "name": "Ross 1057",
+ "pos_x": -32.3125,
+ "pos_y": 26.1875,
+ "pos_z": -12.4375,
+ "stations": "Curtis Outpost,Wang Estate,Parsons Settlement"
+ },
+ {
+ "name": "Ross 1067",
+ "pos_x": -137.4375,
+ "pos_y": 26.03125,
+ "pos_z": 31.71875,
+ "stations": "Whitson Survey,Lousma Mines,Rosenberg Horizons"
+ },
+ {
+ "name": "Ross 1069",
+ "pos_x": -50.53125,
+ "pos_y": 10.625,
+ "pos_z": 1.03125,
+ "stations": "Baudry Gateway,Crown Hub,Viehbock City,Blaschke Penal colony,Still Terminal"
+ },
+ {
+ "name": "Ross 112",
+ "pos_x": -28,
+ "pos_y": 64.28125,
+ "pos_z": -33.34375,
+ "stations": "Egan Orbital,Quick Station,Mitchell Vision"
+ },
+ {
+ "name": "Ross 113",
+ "pos_x": -41.78125,
+ "pos_y": 95.53125,
+ "pos_z": -47.5625,
+ "stations": "Tasman Terminal,Remek Bastion"
+ },
+ {
+ "name": "Ross 115",
+ "pos_x": 17.34375,
+ "pos_y": 50.96875,
+ "pos_z": -7.4375,
+ "stations": "Planck Orbital,Eudoxus Works"
+ },
+ {
+ "name": "Ross 128",
+ "pos_x": 5.53125,
+ "pos_y": 9.4375,
+ "pos_z": 0.125,
+ "stations": "Galton Hangar,Warren Prison Mine"
+ },
+ {
+ "name": "Ross 129",
+ "pos_x": 41.9375,
+ "pos_y": 74.65625,
+ "pos_z": 3.4375,
+ "stations": "Yang Hub,Berezovoy Orbital,Newman Station,Bretnor Base,Wheelock Laboratory"
+ },
+ {
+ "name": "Ross 130",
+ "pos_x": -3.90625,
+ "pos_y": 41,
+ "pos_z": 19.75,
+ "stations": "Crippen Station,Hope City,Landsteiner Point,Ray Port,Daniel Landing"
+ },
+ {
+ "name": "Ross 140",
+ "pos_x": -83.3125,
+ "pos_y": 11.15625,
+ "pos_z": 102.1875,
+ "stations": "Huberath Orbital,Soukup Hub,Reed Hub"
+ },
+ {
+ "name": "Ross 145",
+ "pos_x": -31.5625,
+ "pos_y": 9.90625,
+ "pos_z": 17.3125,
+ "stations": "Levchenko City,Musa al-Khwarizmi Base,Simpson Gateway,Hennen City"
+ },
+ {
+ "name": "Ross 151",
+ "pos_x": -89.03125,
+ "pos_y": 9.90625,
+ "pos_z": 94.21875,
+ "stations": "Walker Ring,Kuhn Gateway,Wnuk-Lipinski's Folly,Marley Mine,Baudin's Folly"
+ },
+ {
+ "name": "Ross 154",
+ "pos_x": -1.9375,
+ "pos_y": -1.84375,
+ "pos_z": 9.3125,
+ "stations": "Birkeland City,Elder Works,Blaschke's Folly"
+ },
+ {
+ "name": "Ross 156",
+ "pos_x": -25.40625,
+ "pos_y": -22.15625,
+ "pos_z": 107.5,
+ "stations": "Bohm Landing"
+ },
+ {
+ "name": "Ross 162",
+ "pos_x": -26.25,
+ "pos_y": -28.71875,
+ "pos_z": 87.34375,
+ "stations": "Jun Settlement,Jacquard Port,Womack Point"
+ },
+ {
+ "name": "Ross 163",
+ "pos_x": -64.25,
+ "pos_y": 8,
+ "pos_z": 34,
+ "stations": "Norton Depot,Tarelkin City,Reiter Horizons"
+ },
+ {
+ "name": "Ross 193",
+ "pos_x": -34.6875,
+ "pos_y": -29.25,
+ "pos_z": 35.625,
+ "stations": "Hopkins Terminal,Hooke Platform,Collins Base"
+ },
+ {
+ "name": "Ross 199",
+ "pos_x": -101.96875,
+ "pos_y": 0.96875,
+ "pos_z": -11.78125,
+ "stations": "Nicollet Terminal,Kroehl Orbital,Cowper Survey"
+ },
+ {
+ "name": "Ross 209",
+ "pos_x": -48.34375,
+ "pos_y": -69.5625,
+ "pos_z": 49.21875,
+ "stations": "Sturckow Depot,Lee Settlement,Janes Terminal,Margulies Mines,Szentmartony Terminal"
+ },
+ {
+ "name": "Ross 210",
+ "pos_x": -73.5,
+ "pos_y": 10.0625,
+ "pos_z": -15.71875,
+ "stations": "Chebyshev Ring,Fu City,Tokubei Ring,McQuay Lab,Fernandes de Queiros Dock,Swift Gateway,Clauss Gateway,Kendrick Arsenal,Quinn Dock,Lanier Orbital,Lunan Dock"
+ },
+ {
+ "name": "Ross 211",
+ "pos_x": -123.71875,
+ "pos_y": 17.3125,
+ "pos_z": -30.21875,
+ "stations": "Otiman Station"
+ },
+ {
+ "name": "Ross 223",
+ "pos_x": -134.0625,
+ "pos_y": -29.0625,
+ "pos_z": -27.0625,
+ "stations": "Woolley Port"
+ },
+ {
+ "name": "Ross 23",
+ "pos_x": -45.71875,
+ "pos_y": 0.875,
+ "pos_z": -75.21875,
+ "stations": "Cady Enterprise,Holub Ring,Snodgrass Enterprise,Acharya Gateway,Rowley Vision"
+ },
+ {
+ "name": "Ross 249",
+ "pos_x": -48.78125,
+ "pos_y": -11.625,
+ "pos_z": -20.5625,
+ "stations": "Alvarez de Pineda Freeport,Farmer's Inheritance,Stevens Depot"
+ },
+ {
+ "name": "Ross 257",
+ "pos_x": -56.9375,
+ "pos_y": -16.09375,
+ "pos_z": 26.90625,
+ "stations": "Tokarev Holdings,Macomb Beacon,Gauss Holdings"
+ },
+ {
+ "name": "Ross 263",
+ "pos_x": -54.375,
+ "pos_y": -28.3125,
+ "pos_z": 13.375,
+ "stations": "Coats Terminal,Doi City,Volta Gateway,Neumann Survey,Scott Penal colony,Fulton Station,Vardeman Observatory,Buffett Hub"
+ },
+ {
+ "name": "Ross 274",
+ "pos_x": -138.96875,
+ "pos_y": -78.65625,
+ "pos_z": 18,
+ "stations": "Goulart City,Ostrander Penal colony,Haldeman II Vision"
+ },
+ {
+ "name": "Ross 279",
+ "pos_x": -34.09375,
+ "pos_y": -41.875,
+ "pos_z": 14.3125,
+ "stations": "Swift Landing,Resnick Legacy"
+ },
+ {
+ "name": "Ross 298",
+ "pos_x": -59.8125,
+ "pos_y": -42,
+ "pos_z": -12.625,
+ "stations": "Pelt's Progress,Henize City,Wilcutt Colony"
+ },
+ {
+ "name": "Ross 302",
+ "pos_x": -39.1875,
+ "pos_y": -2.15625,
+ "pos_z": -15.78125,
+ "stations": "Avdeyev Dock,Jendrassik Station"
+ },
+ {
+ "name": "Ross 310",
+ "pos_x": -80.65625,
+ "pos_y": -6.21875,
+ "pos_z": -43,
+ "stations": "Morey Port"
+ },
+ {
+ "name": "Ross 311",
+ "pos_x": -63.375,
+ "pos_y": -92.59375,
+ "pos_z": -35.5625,
+ "stations": "Leestma Orbital,Jones Laboratory"
+ },
+ {
+ "name": "Ross 318",
+ "pos_x": -22,
+ "pos_y": 4.25,
+ "pos_z": -14.875,
+ "stations": "Herreshoff's Pride,Noon Escape"
+ },
+ {
+ "name": "Ross 33",
+ "pos_x": 0.1875,
+ "pos_y": -8.78125,
+ "pos_z": -28.96875,
+ "stations": "Crown City,Jordan Market"
+ },
+ {
+ "name": "Ross 332",
+ "pos_x": -43.625,
+ "pos_y": -23.15625,
+ "pos_z": -74.125,
+ "stations": "Lawrence Ring,Pettit Orbital,Garan Hub"
+ },
+ {
+ "name": "Ross 335",
+ "pos_x": -58.625,
+ "pos_y": -49.78125,
+ "pos_z": -129.40625,
+ "stations": "McHugh Observatory"
+ },
+ {
+ "name": "Ross 340",
+ "pos_x": -67.875,
+ "pos_y": -14.125,
+ "pos_z": -93.84375,
+ "stations": "Casper Port,Nyberg Market,Bowen Terminal,Laveykin Terminal,Bulychev Camp,Navigator Point,Reilly Laboratory,Planck Hub,Slayton Penal colony"
+ },
+ {
+ "name": "Ross 342",
+ "pos_x": -67.8125,
+ "pos_y": -12.21875,
+ "pos_z": -97.3125,
+ "stations": "Gilliland Station,Chilton Port,Nicollet Landing,Offutt's Claim"
+ },
+ {
+ "name": "Ross 345",
+ "pos_x": -54.375,
+ "pos_y": -6.75,
+ "pos_z": -75.6875,
+ "stations": "Tudela Enterprise,Bakerloo"
+ },
+ {
+ "name": "Ross 346",
+ "pos_x": -33.5,
+ "pos_y": -8.25,
+ "pos_z": -49.59375,
+ "stations": "Mendel Settlement,Carrier Platform"
+ },
+ {
+ "name": "Ross 364",
+ "pos_x": -67.03125,
+ "pos_y": -5.15625,
+ "pos_z": -81.21875,
+ "stations": "Nourse Hub,Montrose Orbital"
+ },
+ {
+ "name": "Ross 370",
+ "pos_x": -29.28125,
+ "pos_y": 0.75,
+ "pos_z": -36.6875,
+ "stations": "Nusslein-Volhard Station,Ansari Terminal,Bacon Dock"
+ },
+ {
+ "name": "Ross 376",
+ "pos_x": -28,
+ "pos_y": -55.65625,
+ "pos_z": -103.6875,
+ "stations": "Tilman Oasis,Clauss Mines"
+ },
+ {
+ "name": "Ross 389",
+ "pos_x": 16.03125,
+ "pos_y": -29.84375,
+ "pos_z": -132.625,
+ "stations": "Caillie Dock,Quick Settlement,Holdstock Installation"
+ },
+ {
+ "name": "Ross 409",
+ "pos_x": -3.1875,
+ "pos_y": -3.15625,
+ "pos_z": -121.21875,
+ "stations": "Shepard Hub,Peano Orbital,Quaglia Hub,Bering Ring,Simmons Orbital,Hambly Gateway,Nobel Station,Williams City,Shepard Port,Dunyach Bastion,Bulychev Landing,Sturt Camp"
+ },
+ {
+ "name": "Ross 41",
+ "pos_x": 7.09375,
+ "pos_y": -6.5,
+ "pos_z": -27.09375,
+ "stations": "Ham Dock,Joule Relay,Laird Survey"
+ },
+ {
+ "name": "Ross 411",
+ "pos_x": -0.34375,
+ "pos_y": -3.53125,
+ "pos_z": -110.90625,
+ "stations": "McCulley Platform"
+ },
+ {
+ "name": "Ross 42",
+ "pos_x": 10.625,
+ "pos_y": -8.75,
+ "pos_z": -39.40625,
+ "stations": "Pythagoras Dock,Bartoe Station,Nasmyth Enterprise,Clark Hub,Gaffney Port,Bryant Vista"
+ },
+ {
+ "name": "Ross 429",
+ "pos_x": 56.25,
+ "pos_y": 1.78125,
+ "pos_z": -28.96875,
+ "stations": "Al-Battani Enterprise,Clark Enterprise,DeLucas Barracks,Adams Base,Mukai Gateway"
+ },
+ {
+ "name": "Ross 437",
+ "pos_x": 115.53125,
+ "pos_y": 62.46875,
+ "pos_z": -52.78125,
+ "stations": "Conway Terminal,Currie Station,Celebi's Folly"
+ },
+ {
+ "name": "Ross 440",
+ "pos_x": 26.75,
+ "pos_y": 14.8125,
+ "pos_z": -11.375,
+ "stations": "Budrys Silo,Carter Hub,Ramsay Hub"
+ },
+ {
+ "name": "Ross 444",
+ "pos_x": 106.09375,
+ "pos_y": 79.5625,
+ "pos_z": -40.28125,
+ "stations": "Chretien Terminal,Crouch Dock,Bushnell Settlement,Fontenay Vision"
+ },
+ {
+ "name": "Ross 446",
+ "pos_x": 14.21875,
+ "pos_y": 16.90625,
+ "pos_z": -6.53125,
+ "stations": "Song Plant,Ingstad Installation"
+ },
+ {
+ "name": "Ross 447",
+ "pos_x": -36.5,
+ "pos_y": 52.46875,
+ "pos_z": -38.15625,
+ "stations": "Perga Station,Rangarajan Oasis,Fowler Hub,Scobee Works,Sturt Depot,Resnick Beacon,Carpini Vision,Fourneyron Installation,Alpers Horizons"
+ },
+ {
+ "name": "Ross 467",
+ "pos_x": 44.46875,
+ "pos_y": 66.15625,
+ "pos_z": 42.03125,
+ "stations": "Bennett Hub,Sharma Terminal,Pribylov Arena,Bulgarin Penal colony,Kapp Prospect,Coulomb Installation,Klein Escape"
+ },
+ {
+ "name": "Ross 47",
+ "pos_x": 4.53125,
+ "pos_y": -2.875,
+ "pos_z": -18.25,
+ "stations": "Gidzenko Prospect,Volynov Hub,Molina Platform"
+ },
+ {
+ "name": "Ross 478",
+ "pos_x": 24.75,
+ "pos_y": 52.34375,
+ "pos_z": 31.46875,
+ "stations": "Carson Hub,Blenkinsop Dock"
+ },
+ {
+ "name": "Ross 484",
+ "pos_x": 39.75,
+ "pos_y": 96.75,
+ "pos_z": 43.375,
+ "stations": "Hackworth City"
+ },
+ {
+ "name": "Ross 486",
+ "pos_x": 14.65625,
+ "pos_y": 39.46875,
+ "pos_z": 18.90625,
+ "stations": "Teng-hui Terminal,Johnson Barracks,King Observatory,Usachov Plant"
+ },
+ {
+ "name": "Ross 490",
+ "pos_x": 3.59375,
+ "pos_y": 23.5,
+ "pos_z": 7.53125,
+ "stations": "Citi Dock,Dunyach Enterprise,Griffiths Dock"
+ },
+ {
+ "name": "Ross 491",
+ "pos_x": 18.15625,
+ "pos_y": 103.9375,
+ "pos_z": 39.4375,
+ "stations": "Ozanne Station,Cabral Settlement,Farouk Platform,Pohl Survey"
+ },
+ {
+ "name": "Ross 494",
+ "pos_x": -18,
+ "pos_y": 129.4375,
+ "pos_z": 32.1875,
+ "stations": "Narita Terminal,Bisson Prospect"
+ },
+ {
+ "name": "Ross 495",
+ "pos_x": -36.71875,
+ "pos_y": 153.875,
+ "pos_z": 34.96875,
+ "stations": "Citi Mines"
+ },
+ {
+ "name": "Ross 5",
+ "pos_x": -40.8125,
+ "pos_y": -17.75,
+ "pos_z": -20.40625,
+ "stations": "Neumann Penal colony,Ham Colony"
+ },
+ {
+ "name": "Ross 514",
+ "pos_x": -38.40625,
+ "pos_y": 109.34375,
+ "pos_z": 94.0625,
+ "stations": "Ahmed Settlement,Morrow Prospect"
+ },
+ {
+ "name": "Ross 523",
+ "pos_x": -60.78125,
+ "pos_y": 67.15625,
+ "pos_z": 22.34375,
+ "stations": "Mendeleev Platform,Matteucci Point,Scalzi Horizons"
+ },
+ {
+ "name": "Ross 528",
+ "pos_x": 5.21875,
+ "pos_y": 58.15625,
+ "pos_z": 145.6875,
+ "stations": "Drzewiecki Hub,Forfait Terminal,Hassanein Depot"
+ },
+ {
+ "name": "Ross 529",
+ "pos_x": 4.71875,
+ "pos_y": 18.5625,
+ "pos_z": 56.40625,
+ "stations": "Dzhanibekov Beacon"
+ },
+ {
+ "name": "Ross 545",
+ "pos_x": -104.53125,
+ "pos_y": -106.875,
+ "pos_z": -90.78125,
+ "stations": "Noether Settlement,Staden's Progress"
+ },
+ {
+ "name": "Ross 546",
+ "pos_x": -85,
+ "pos_y": -35.34375,
+ "pos_z": -67.65625,
+ "stations": "Kubasov Horizons,Bunch Survey"
+ },
+ {
+ "name": "Ross 562",
+ "pos_x": -62.21875,
+ "pos_y": 5.71875,
+ "pos_z": -81.28125,
+ "stations": "Hackworth Dock"
+ },
+ {
+ "name": "Ross 569",
+ "pos_x": 13.75,
+ "pos_y": -111.96875,
+ "pos_z": -96.4375,
+ "stations": "Popov Hub,Pimi Dock"
+ },
+ {
+ "name": "Ross 584",
+ "pos_x": 10.9375,
+ "pos_y": -33.65625,
+ "pos_z": -36.4375,
+ "stations": "Wrangell Dock,Pythagoras Beacon,Shriver Station,Carey Ring"
+ },
+ {
+ "name": "Ross 588",
+ "pos_x": 4,
+ "pos_y": -33.40625,
+ "pos_z": -43.46875,
+ "stations": "Koch Platform,Hopkins Landing"
+ },
+ {
+ "name": "Ross 591",
+ "pos_x": -32.1875,
+ "pos_y": -28.09375,
+ "pos_z": -111.375,
+ "stations": "Popper Terminal"
+ },
+ {
+ "name": "Ross 596",
+ "pos_x": -31.25,
+ "pos_y": -21.46875,
+ "pos_z": -134.59375,
+ "stations": "Ehrlich Dock,Potter Dock,Resnik Market,Wiley Enterprise,Bobko Orbital,Tryggvason Ring"
+ },
+ {
+ "name": "Ross 597",
+ "pos_x": -14.125,
+ "pos_y": -37.40625,
+ "pos_z": -111.09375,
+ "stations": "Malyshev Vision,Fiennes Survey"
+ },
+ {
+ "name": "Ross 598",
+ "pos_x": -14.65625,
+ "pos_y": -38.125,
+ "pos_z": -131.6875,
+ "stations": "McMullen Landing,Garden Vision"
+ },
+ {
+ "name": "Ross 601",
+ "pos_x": 16.625,
+ "pos_y": -20.75,
+ "pos_z": -141.71875,
+ "stations": "Tarski Terminal,Ayerdhal Base"
+ },
+ {
+ "name": "Ross 609",
+ "pos_x": 76.3125,
+ "pos_y": 10.34375,
+ "pos_z": -155.25,
+ "stations": "Ross Dock"
+ },
+ {
+ "name": "Ross 611",
+ "pos_x": 60.875,
+ "pos_y": 18.875,
+ "pos_z": -144.6875,
+ "stations": "Bridger Dock,Viete Plant"
+ },
+ {
+ "name": "Ross 623",
+ "pos_x": 41.65625,
+ "pos_y": 30.75,
+ "pos_z": -38.53125,
+ "stations": "Meitner Landing"
+ },
+ {
+ "name": "Ross 625",
+ "pos_x": 42.46875,
+ "pos_y": 32.78125,
+ "pos_z": -39.375,
+ "stations": "Swanson Outpost,Mohri Platform"
+ },
+ {
+ "name": "Ross 632",
+ "pos_x": 53,
+ "pos_y": 114.34375,
+ "pos_z": 2.8125,
+ "stations": "Williams Hub,Carpini Port,Weyn Landing"
+ },
+ {
+ "name": "Ross 640",
+ "pos_x": -31.6875,
+ "pos_y": 35.125,
+ "pos_z": 18.9375,
+ "stations": "Orbital Stockade N-65"
+ },
+ {
+ "name": "Ross 643",
+ "pos_x": -48.8125,
+ "pos_y": 71.15625,
+ "pos_z": 112.0625,
+ "stations": "Kotzebue Prospect"
+ },
+ {
+ "name": "Ross 646",
+ "pos_x": -80.9375,
+ "pos_y": 60.75,
+ "pos_z": 115,
+ "stations": "McArthur Enterprise,Carey Port,Ali Terminal,Cochrane Settlement"
+ },
+ {
+ "name": "Ross 651",
+ "pos_x": -71.0625,
+ "pos_y": -9.625,
+ "pos_z": 91.15625,
+ "stations": "Fuca Base,Santos Survey,Webb Landing,Serling Landing"
+ },
+ {
+ "name": "Ross 665",
+ "pos_x": -127.65625,
+ "pos_y": -6.375,
+ "pos_z": -22.75,
+ "stations": "Mills Station,Malpighi Oasis,Wetherbee Hub"
+ },
+ {
+ "name": "Ross 667",
+ "pos_x": -170.53125,
+ "pos_y": 5.71875,
+ "pos_z": -49.1875,
+ "stations": "Alexander Terminal,Naddoddur Port,Back Hub"
+ },
+ {
+ "name": "Ross 671",
+ "pos_x": -17.53125,
+ "pos_y": -13.84375,
+ "pos_z": 0.625,
+ "stations": "Akiyama Hub,Thomson Terminal,Watson Port,Matheson Terminal,Verrazzano Point,Phillips Works,Heisenberg Orbital,Lebedev Dock,Napier Installation"
+ },
+ {
+ "name": "Ross 680",
+ "pos_x": -57.71875,
+ "pos_y": -41.125,
+ "pos_z": -26.625,
+ "stations": "Pelliot Plant,Navigator Plant"
+ },
+ {
+ "name": "Ross 682",
+ "pos_x": 47.53125,
+ "pos_y": 40.46875,
+ "pos_z": -53.875,
+ "stations": "Popov City,Gibson Station,Diamond Station,North Landing,Heaviside Depot"
+ },
+ {
+ "name": "Ross 685",
+ "pos_x": 87.3125,
+ "pos_y": 75.3125,
+ "pos_z": -87.84375,
+ "stations": "Barry Works,Shukor Colony,Makeev Works"
+ },
+ {
+ "name": "Ross 692",
+ "pos_x": 64.0625,
+ "pos_y": 63.625,
+ "pos_z": 28.5625,
+ "stations": "Lorenz Port,Massimino Station,Altman Station,Phillpotts Installation,Birmingham Landing"
+ },
+ {
+ "name": "Ross 695",
+ "pos_x": 18.8125,
+ "pos_y": 20.03125,
+ "pos_z": 8.78125,
+ "stations": "Bresnik Orbital,Brunner Observatory,Hawley Hub,Feoktistov Orbital,Palmer Silo"
+ },
+ {
+ "name": "Ross 697",
+ "pos_x": 55.96875,
+ "pos_y": 63.125,
+ "pos_z": 27.21875,
+ "stations": "Lousma Relay,Bunsen's Claim"
+ },
+ {
+ "name": "Ross 705",
+ "pos_x": 74.59375,
+ "pos_y": 90.03125,
+ "pos_z": 49.1875,
+ "stations": "Ejeta City,Serling Installation,Hartsfield Dock,Grandin Gateway"
+ },
+ {
+ "name": "Ross 706",
+ "pos_x": -79.09375,
+ "pos_y": 32.28125,
+ "pos_z": 57.09375,
+ "stations": "Galvani Colony,Shatalov Dock,Zalyotin Terminal,Fossey Settlement,Cook Works"
+ },
+ {
+ "name": "Ross 708",
+ "pos_x": -35.6875,
+ "pos_y": 14.0625,
+ "pos_z": 24.125,
+ "stations": "Eyharts Orbital,Smith Station,Rominger Plant"
+ },
+ {
+ "name": "Ross 709",
+ "pos_x": -54.625,
+ "pos_y": 19.65625,
+ "pos_z": 41.34375,
+ "stations": "Reisman Settlement"
+ },
+ {
+ "name": "Ross 71",
+ "pos_x": -3.375,
+ "pos_y": 16.15625,
+ "pos_z": -119.96875,
+ "stations": "Hopkins Terminal"
+ },
+ {
+ "name": "Ross 718",
+ "pos_x": -51.0625,
+ "pos_y": -7.75,
+ "pos_z": 93.3125,
+ "stations": "Bamford Enterprise,Nicollier Ring,Lenoir Hub,Pawelczyk Depot,Birkeland Enterprise,Bain Enterprise,Frick Gateway,Nye City,Pauli Terminal,Sullivan Orbital,Santos Plant,Tolstoi Relay,Rutherford Penal colony"
+ },
+ {
+ "name": "Ross 719",
+ "pos_x": -41.25,
+ "pos_y": -5.40625,
+ "pos_z": 98.53125,
+ "stations": "Christian Station,Gordon Holdings"
+ },
+ {
+ "name": "Ross 720",
+ "pos_x": -18.375,
+ "pos_y": -4.09375,
+ "pos_z": 49.90625,
+ "stations": "Raleigh Orbital,Roberts Enterprise,Linge Hub,Narita's Inheritance"
+ },
+ {
+ "name": "Ross 725",
+ "pos_x": -72.21875,
+ "pos_y": -26.1875,
+ "pos_z": 141.46875,
+ "stations": "Stairs Reach"
+ },
+ {
+ "name": "Ross 730",
+ "pos_x": -22.25,
+ "pos_y": 2.71875,
+ "pos_z": 16.375,
+ "stations": "Drebbel City,Pavlov Settlement,Chadwick Prospect"
+ },
+ {
+ "name": "Ross 732",
+ "pos_x": -95.84375,
+ "pos_y": 12.21875,
+ "pos_z": 62.09375,
+ "stations": "Minkowski Enterprise,Mackenzie Survey,Dowling Station"
+ },
+ {
+ "name": "Ross 733",
+ "pos_x": -49.625,
+ "pos_y": 3.625,
+ "pos_z": 37.3125,
+ "stations": "Forest Terminal,Strekalov Gateway,Schottky Station,Serebrov Silo"
+ },
+ {
+ "name": "Ross 750",
+ "pos_x": -110.1875,
+ "pos_y": -9.96875,
+ "pos_z": 94.375,
+ "stations": "Holdstock Hub"
+ },
+ {
+ "name": "Ross 752",
+ "pos_x": -116.90625,
+ "pos_y": -37.875,
+ "pos_z": 101.3125,
+ "stations": "Grant's Claim,Amnuel Base"
+ },
+ {
+ "name": "Ross 754",
+ "pos_x": -68.4375,
+ "pos_y": -17.59375,
+ "pos_z": 47.78125,
+ "stations": "Roberts Hub,Dana Gateway,Bardeen Platform,Northrop Orbital"
+ },
+ {
+ "name": "Ross 765",
+ "pos_x": -72.78125,
+ "pos_y": 7,
+ "pos_z": -1.90625,
+ "stations": "Melroy Port,Olivas Penal colony,Pogue Platform"
+ },
+ {
+ "name": "Ross 769",
+ "pos_x": -26.34375,
+ "pos_y": -38.09375,
+ "pos_z": 42.03125,
+ "stations": "Rennie Platform"
+ },
+ {
+ "name": "Ross 773",
+ "pos_x": -59.09375,
+ "pos_y": -22.09375,
+ "pos_z": 21.3125,
+ "stations": "Brooks Base,McCormick Survey,Hornby Vision"
+ },
+ {
+ "name": "Ross 775",
+ "pos_x": -18.8125,
+ "pos_y": -8.90625,
+ "pos_z": 6.78125,
+ "stations": "Harvey Port,Gamow Gateway,Ivanchenkov Enterprise,Humphreys Survey,Aikin Landing"
+ },
+ {
+ "name": "Ross 777",
+ "pos_x": -130.90625,
+ "pos_y": -31.9375,
+ "pos_z": 24.8125,
+ "stations": "Bradley Dock,Kerr Vision,Fozard Dock,Galois Depot,Littlewood Dock,King Silo"
+ },
+ {
+ "name": "Ross 779",
+ "pos_x": -59.46875,
+ "pos_y": -46.5,
+ "pos_z": 30.84375,
+ "stations": "Carpini Enterprise,Arnold Settlement,Fraas Camp"
+ },
+ {
+ "name": "Ross 780",
+ "pos_x": -6.125,
+ "pos_y": -13.28125,
+ "pos_z": 4.5625,
+ "stations": "Patsayev Penal colony,Aristotle Gateway,Fossum Terminal,Acton Ring"
+ },
+ {
+ "name": "Ross 788",
+ "pos_x": -49.5,
+ "pos_y": -51.75,
+ "pos_z": -45.15625,
+ "stations": "Goran Gmitrovic,Chwedyk Ring,Brookes Abyss,Leinster Landing"
+ },
+ {
+ "name": "Ross 809",
+ "pos_x": -71.78125,
+ "pos_y": 77.78125,
+ "pos_z": 51.09375,
+ "stations": "Braun City"
+ },
+ {
+ "name": "Ross 812",
+ "pos_x": -40.625,
+ "pos_y": 40.875,
+ "pos_z": 24.65625,
+ "stations": "Fraas Plant"
+ },
+ {
+ "name": "Ross 835",
+ "pos_x": 3.21875,
+ "pos_y": 63.84375,
+ "pos_z": 24.125,
+ "stations": "Dobrovolskiy City,Hoshide Horizons,Joule Enterprise,Dalmas Base"
+ },
+ {
+ "name": "Ross 842",
+ "pos_x": 43.78125,
+ "pos_y": 99.9375,
+ "pos_z": 78.90625,
+ "stations": "Baker Enterprise,Solovyev Park,Vela Dock,Bernoulli Depot"
+ },
+ {
+ "name": "Ross 847",
+ "pos_x": 16.5,
+ "pos_y": 54.03125,
+ "pos_z": 42.875,
+ "stations": "Houtman Dock,Popov Orbital,Monge Holdings,Lee Station,Wells Penal colony"
+ },
+ {
+ "name": "Ross 85",
+ "pos_x": 17.375,
+ "pos_y": 25.5,
+ "pos_z": -19.875,
+ "stations": "Mohmand Station,Kimbrough Penal colony,Lyell Ring"
+ },
+ {
+ "name": "Ross 853",
+ "pos_x": 35.625,
+ "pos_y": 31.40625,
+ "pos_z": 123.1875,
+ "stations": "Weyl Orbital,Webb Port,Froude Orbital,Darnielle Dock,Ray Terminal,Linaweaver Station"
+ },
+ {
+ "name": "Ross 860",
+ "pos_x": -20.125,
+ "pos_y": 19.28125,
+ "pos_z": 19,
+ "stations": "Wedge Hub,Hadamard Terminal,Sullivan Port,Rennie Arsenal,Cavendish Lab,Hamilton Holdings,Hertz Point"
+ },
+ {
+ "name": "Ross 878",
+ "pos_x": 17.53125,
+ "pos_y": 19.5625,
+ "pos_z": -57.375,
+ "stations": "Jakes Enterprise,Farrukh Prospect,Wells Ring,Atwood Depot"
+ },
+ {
+ "name": "Ross 89",
+ "pos_x": 23.1875,
+ "pos_y": 51.96875,
+ "pos_z": -43.40625,
+ "stations": "Xiaoguan Dock,Burnell Terminal,Hawking Terminal,Faris Enterprise,Hoard Hub,Whitworth Gateway,Humphreys Silo"
+ },
+ {
+ "name": "Ross 898",
+ "pos_x": 88.3125,
+ "pos_y": 112.15625,
+ "pos_z": -25.0625,
+ "stations": "Wang Horizons,Usachov Survey,Lloyd Horizons"
+ },
+ {
+ "name": "Ross 905",
+ "pos_x": 4.46875,
+ "pos_y": 31.9375,
+ "pos_z": -7.21875,
+ "stations": "Jenner Refinery,Sturckow Port,Weber Legacy"
+ },
+ {
+ "name": "Ross 911",
+ "pos_x": 45.25,
+ "pos_y": 87.71875,
+ "pos_z": -4.53125,
+ "stations": "Goeppert-Mayer Base,McKay Dock,Glazkov Keep"
+ },
+ {
+ "name": "Ross 917",
+ "pos_x": -28.84375,
+ "pos_y": 118.75,
+ "pos_z": -32.78125,
+ "stations": "Laird Settlement,Stokes Settlement,Ehrenfried Kegel Settlement"
+ },
+ {
+ "name": "Ross 920",
+ "pos_x": 74.28125,
+ "pos_y": 123.75,
+ "pos_z": 12.4375,
+ "stations": "McMonagle Hub"
+ },
+ {
+ "name": "Ross 922",
+ "pos_x": 83.5,
+ "pos_y": 123.21875,
+ "pos_z": 19.78125,
+ "stations": "Cayley Platform,Elder Colony"
+ },
+ {
+ "name": "Ross 93",
+ "pos_x": 11.46875,
+ "pos_y": 34.5625,
+ "pos_z": -27.6875,
+ "stations": "Garratt Port,Virts Dock,Fraley Town,Tennyson d'Eyncourt Terminal"
+ },
+ {
+ "name": "Ross 931",
+ "pos_x": 80.46875,
+ "pos_y": 120.59375,
+ "pos_z": 24.53125,
+ "stations": "Vishweswarayya Dock"
+ },
+ {
+ "name": "Ross 94",
+ "pos_x": 27.4375,
+ "pos_y": 80.375,
+ "pos_z": -62.40625,
+ "stations": "Kingsbury Terminal,Galindo Penal colony,Fremont Holdings"
+ },
+ {
+ "name": "Ross 970",
+ "pos_x": 59.96875,
+ "pos_y": 110.375,
+ "pos_z": 43,
+ "stations": "Poleshchuk Landing,Teng-hui Camp"
+ },
+ {
+ "name": "Ross 98",
+ "pos_x": 44.1875,
+ "pos_y": 63.65625,
+ "pos_z": -27.4375,
+ "stations": "Foale Enterprise,Leestma City,Brunel Ring,Behnken Observatory,Gold Arsenal"
+ },
+ {
+ "name": "Ross 986",
+ "pos_x": -0.25,
+ "pos_y": 7.1875,
+ "pos_z": -19.25,
+ "stations": "Jones Station,Nakasone Terminal,Shepard Co-operative,Howe Vista"
+ },
+ {
+ "name": "Ross 991",
+ "pos_x": -18.65625,
+ "pos_y": 64.4375,
+ "pos_z": -12.59375,
+ "stations": "Mallett Depot,Asire Dock,Lee Penal colony"
+ },
+ {
+ "name": "Rotanev",
+ "pos_x": -83.4375,
+ "pos_y": -27.84375,
+ "pos_z": 49.40625,
+ "stations": "Ride Ring,Andreas City,Smith Lab,Schottky Silo"
+ },
+ {
+ "name": "Roustem",
+ "pos_x": 5.8125,
+ "pos_y": -44.96875,
+ "pos_z": -82.59375,
+ "stations": "Meikle Terminal,Solovyov Settlement,Kovalyonok Outpost"
+ },
+ {
+ "name": "Roustet",
+ "pos_x": 163.8125,
+ "pos_y": -138.1875,
+ "pos_z": 3.09375,
+ "stations": "Zhukovsky Orbital,Gaspar de Lemos' Inheritance,Ortiz Moreno Colony"
+ },
+ {
+ "name": "Roxo",
+ "pos_x": -107.3125,
+ "pos_y": 51.40625,
+ "pos_z": -49.59375,
+ "stations": "Flynn Silo,Moore Refinery"
+ },
+ {
+ "name": "RR Caeli",
+ "pos_x": 18.3125,
+ "pos_y": -18.59375,
+ "pos_z": -4.6875,
+ "stations": "Rennie City,Hooke City,Herreshoff Installation"
+ },
+ {
+ "name": "RST 1154",
+ "pos_x": -20,
+ "pos_y": -90.90625,
+ "pos_z": 35.5625,
+ "stations": "Chretien Gateway,Kavandi Terminal,Sellers Port"
+ },
+ {
+ "name": "Ru Shil",
+ "pos_x": 103.78125,
+ "pos_y": -59.125,
+ "pos_z": 38.15625,
+ "stations": "McHugh Vision,Harrison Landing,Aller Dock,Langley Hub,Bok Orbital,Safdie Terminal,Michell Beacon,Babcock Dock,Riazuddin Gateway,Argelander Station"
+ },
+ {
+ "name": "Ru Shui",
+ "pos_x": 44.21875,
+ "pos_y": 63.1875,
+ "pos_z": 134.6875,
+ "stations": "Resnick Asylum"
+ },
+ {
+ "name": "Ru Shun",
+ "pos_x": 125.9375,
+ "pos_y": -182.09375,
+ "pos_z": -62.09375,
+ "stations": "Louis de Lacaille Station,Wales Survey,Parker Mines,Cowling Horizons,Bethke Base"
+ },
+ {
+ "name": "Ruadari",
+ "pos_x": -165.40625,
+ "pos_y": -12.25,
+ "pos_z": -12.21875,
+ "stations": "Diamond Landing,Almagro Works,Galouye Depot"
+ },
+ {
+ "name": "Ruchbah",
+ "pos_x": -78.78125,
+ "pos_y": -3.5625,
+ "pos_z": -60.5625,
+ "stations": "Griffiths Horizons,Feynman Vision,Blish Vision,Elder City,Vernadsky Relay"
+ },
+ {
+ "name": "Rudjer Boskovic",
+ "pos_x": 71.9375,
+ "pos_y": -45.875,
+ "pos_z": -35.75,
+ "stations": "New Belgrade,Merchiston Camp"
+ },
+ {
+ "name": "Rudranbarii",
+ "pos_x": -108.8125,
+ "pos_y": 43.65625,
+ "pos_z": 19.6875,
+ "stations": "Bluford Station,Vittori Gateway,Derleth's Claim"
+ },
+ {
+ "name": "Rudrani",
+ "pos_x": 59.84375,
+ "pos_y": 115.125,
+ "pos_z": -116.65625,
+ "stations": "Levy Beacon,Mieville Works,MacCurdy Base"
+ },
+ {
+ "name": "Rudrato",
+ "pos_x": 140.78125,
+ "pos_y": -9.65625,
+ "pos_z": 59.6875,
+ "stations": "Kelly Dock"
+ },
+ {
+ "name": "Rudrusa",
+ "pos_x": 212.34375,
+ "pos_y": -137.4375,
+ "pos_z": 19.9375,
+ "stations": "Langley Vision,Amis Silo"
+ },
+ {
+ "name": "Rudrushu",
+ "pos_x": -56.90625,
+ "pos_y": -121.375,
+ "pos_z": 30.25,
+ "stations": "Kibalchich Plant,McQuarrie Hub,McQuarrie Horizons,White Installation"
+ },
+ {
+ "name": "Ruga",
+ "pos_x": 115.34375,
+ "pos_y": -103.21875,
+ "pos_z": 101.96875,
+ "stations": "Kludze Dock,Millosevich Colony"
+ },
+ {
+ "name": "Rugabaruwa",
+ "pos_x": 90.84375,
+ "pos_y": -187.34375,
+ "pos_z": -40.1875,
+ "stations": "Wright Landing,Brom Orbital,Archer Enterprise"
+ },
+ {
+ "name": "Rugabugait",
+ "pos_x": 141,
+ "pos_y": 31.375,
+ "pos_z": 55.375,
+ "stations": "Noriega Platform,Yaping Port"
+ },
+ {
+ "name": "Rugabung",
+ "pos_x": 51.25,
+ "pos_y": 22.5625,
+ "pos_z": 146.78125,
+ "stations": "Willis Port"
+ },
+ {
+ "name": "Rugai",
+ "pos_x": -17.28125,
+ "pos_y": -2.65625,
+ "pos_z": 39.40625,
+ "stations": "Nakaya Landing,Wingqvist Reformatory,Foale Installation,Eratosthenes Landing"
+ },
+ {
+ "name": "Ruganike",
+ "pos_x": 67.25,
+ "pos_y": -96.65625,
+ "pos_z": -14.78125,
+ "stations": "Armero Hub,Rosseland Orbital,Olahus Gateway"
+ },
+ {
+ "name": "Rugi",
+ "pos_x": -96.21875,
+ "pos_y": -141.875,
+ "pos_z": 33.59375,
+ "stations": "Fermi Ring,Pauling Station,Virtanen Dock,Fibonacci Enterprise"
+ },
+ {
+ "name": "Rugien",
+ "pos_x": 60.9375,
+ "pos_y": -172.15625,
+ "pos_z": -28.09375,
+ "stations": "Campbell Point,Tarter Port,Ernst Platform"
+ },
+ {
+ "name": "Rugievit",
+ "pos_x": 161.25,
+ "pos_y": -91.6875,
+ "pos_z": 34.78125,
+ "stations": "Belyavsky Works,Jones Barracks"
+ },
+ {
+ "name": "Rugiraochi",
+ "pos_x": 27.75,
+ "pos_y": -55.15625,
+ "pos_z": -123,
+ "stations": "Burckhardt Silo,Coney Point,Evans Colony,Sekowski Penitentiary"
+ },
+ {
+ "name": "Ruhang",
+ "pos_x": 151,
+ "pos_y": -70.6875,
+ "pos_z": 50.1875,
+ "stations": "Harrington Dock,Lewis Station,Henslow Bastion"
+ },
+ {
+ "name": "Ruhang Yeh",
+ "pos_x": 85.65625,
+ "pos_y": -158.25,
+ "pos_z": 104.0625,
+ "stations": "Lindstrand Landing"
+ },
+ {
+ "name": "Ruhanga",
+ "pos_x": 110.3125,
+ "pos_y": -59,
+ "pos_z": 52.78125,
+ "stations": "Hind Station,Lagadha Port,Walter Bastion"
+ },
+ {
+ "name": "Ruhanti",
+ "pos_x": -16.1875,
+ "pos_y": 113,
+ "pos_z": 3.71875,
+ "stations": "Brin Orbital,Diophantus City,Heyerdahl Hub,Jones Vista,Mitchell Point,Farmer Hub,Carleson Station"
+ },
+ {
+ "name": "Ruka",
+ "pos_x": 30.71875,
+ "pos_y": 42.03125,
+ "pos_z": 163.375,
+ "stations": "McArthur Works,Kerwin Platform,Ramsay Asylum"
+ },
+ {
+ "name": "Rukama",
+ "pos_x": 101.40625,
+ "pos_y": -132.25,
+ "pos_z": -77.25,
+ "stations": "Tisserand City,Mayer Station,Kerimov Port,Beaumont's Folly,Stabenow Holdings,Schmidt Enterprise"
+ },
+ {
+ "name": "Rukandins",
+ "pos_x": 56.125,
+ "pos_y": -31.625,
+ "pos_z": 122.6875,
+ "stations": "Mayer Plant"
+ },
+ {
+ "name": "Rukarwadja",
+ "pos_x": 100.90625,
+ "pos_y": -184.125,
+ "pos_z": 39.625,
+ "stations": "Wallerstein Port"
+ },
+ {
+ "name": "Rukminiez",
+ "pos_x": 153.375,
+ "pos_y": -5.25,
+ "pos_z": 49.125,
+ "stations": "White Platform,Rondon City,Sutcliffe Depot"
+ },
+ {
+ "name": "Rukminyinyi",
+ "pos_x": -44.625,
+ "pos_y": 43.5,
+ "pos_z": 124.8125,
+ "stations": "Belyayev Depot,Russell Plant,Cayley Works,Glashow Mines"
+ },
+ {
+ "name": "Run",
+ "pos_x": 22.8125,
+ "pos_y": -165.96875,
+ "pos_z": 34.9375,
+ "stations": "Langley Dock,Smithport,Shepard Point,Brothers Landing,Leif Enterprise"
+ },
+ {
+ "name": "Runab",
+ "pos_x": -18.9375,
+ "pos_y": 39.5,
+ "pos_z": -114.78125,
+ "stations": "Walheim Settlement"
+ },
+ {
+ "name": "Runapa",
+ "pos_x": 108.40625,
+ "pos_y": 3.9375,
+ "pos_z": 102.9375,
+ "stations": "Mattingly Station,Bamford Orbital,Hopper Station,Moseley Vision"
+ },
+ {
+ "name": "Runga",
+ "pos_x": 78.03125,
+ "pos_y": 100.09375,
+ "pos_z": 63.21875,
+ "stations": "Bramah Legacy"
+ },
+ {
+ "name": "Rungu",
+ "pos_x": 0.5625,
+ "pos_y": 58.125,
+ "pos_z": 161.65625,
+ "stations": "Arnarson City,Low Orbital,Payne Dock,Herreshoff Prospect"
+ },
+ {
+ "name": "Runo",
+ "pos_x": 51.125,
+ "pos_y": -155.53125,
+ "pos_z": 44.28125,
+ "stations": "Mitzi's Den,Crossfield Prospect,Libeskind Keep,Hynek Vision,Bob Paffett"
+ },
+ {
+ "name": "Rurema",
+ "pos_x": 79.15625,
+ "pos_y": -157.90625,
+ "pos_z": -27.375,
+ "stations": "Daqing Terminal,Keeler Port,Harvey-Smith Vision,Filter Holdings"
+ },
+ {
+ "name": "Rureri",
+ "pos_x": 86.46875,
+ "pos_y": -51.03125,
+ "pos_z": 0.625,
+ "stations": "Deutsch Terminal,Wilder Landing,van den Hove Beacon"
+ },
+ {
+ "name": "Rusalki",
+ "pos_x": 34.1875,
+ "pos_y": 99.3125,
+ "pos_z": 12.4375,
+ "stations": "Chang-Diaz Base,Ivanishin Terminal"
+ },
+ {
+ "name": "Rusalku",
+ "pos_x": -76.5,
+ "pos_y": -105,
+ "pos_z": 41.875,
+ "stations": "Burckhardt City,Rochon Enterprise,Lopez de Villalobos Arsenal,Darlton Enterprise,Ballard Survey,Pangborn Lab"
+ },
+ {
+ "name": "Rusalkyries",
+ "pos_x": -100.46875,
+ "pos_y": -43.40625,
+ "pos_z": -16.59375,
+ "stations": "Clayton Prospect,Stableford Camp,Pawelczyk Orbital"
+ },
+ {
+ "name": "Rusamai",
+ "pos_x": -36.6875,
+ "pos_y": -22.53125,
+ "pos_z": 145.59375,
+ "stations": "Herreshoff Platform"
+ },
+ {
+ "name": "Rusan",
+ "pos_x": -19.5,
+ "pos_y": -190.4375,
+ "pos_z": -22.5625,
+ "stations": "Harrison Beacon,Babakin Station,Shoemaker Platform"
+ },
+ {
+ "name": "Rusani",
+ "pos_x": -64.75,
+ "pos_y": 57.21875,
+ "pos_z": -82.625,
+ "stations": "Fernandes Market,Houtman Orbital,Mitra Enterprise,Andrews Enterprise,Ray Enterprise,Scithers Orbital,MacLean Enterprise,Cardano Arena,Huberath Terminal,Cowper Hub"
+ },
+ {
+ "name": "Rusasaei",
+ "pos_x": 96.90625,
+ "pos_y": -44.6875,
+ "pos_z": -54.625,
+ "stations": "Duke Arsenal,Qureshi Hub"
+ },
+ {
+ "name": "Rusattra",
+ "pos_x": 63.28125,
+ "pos_y": 43.9375,
+ "pos_z": 141.75,
+ "stations": "Due Mine,Paulo da Gama Asylum"
+ },
+ {
+ "name": "Russa",
+ "pos_x": -118.3125,
+ "pos_y": -80.03125,
+ "pos_z": -52.96875,
+ "stations": "Lee Gateway,Ogden Penal colony,Carr Enterprise,Alvarado Port"
+ },
+ {
+ "name": "Russi Wang",
+ "pos_x": 42.78125,
+ "pos_y": 80.59375,
+ "pos_z": 82.6875,
+ "stations": "Galindo Terminal"
+ },
+ {
+ "name": "Rutena",
+ "pos_x": -46.46875,
+ "pos_y": 35.375,
+ "pos_z": -105.84375,
+ "stations": "Weitz City,McDivitt Settlement,Chu City,Waldrop Palace,Szameit's Inheritance"
+ },
+ {
+ "name": "Rutera",
+ "pos_x": 31.03125,
+ "pos_y": -134.9375,
+ "pos_z": -78.6875,
+ "stations": "Mikoyan Port,Shoemaker Settlement"
+ },
+ {
+ "name": "Rutu",
+ "pos_x": -16.625,
+ "pos_y": 15.40625,
+ "pos_z": 133.375,
+ "stations": "Morin Orbital,Yeliseyev Enterprise,Resnik Dock,Ito Hub,Disch Town,Garriott Station,Edgeworth Hub,Linge Keep,Cormack Orbital,Stasheff Exchange,Smith Penal colony,Agassiz Port,Khayyam Enterprise"
+ },
+ {
+ "name": "Rutujil",
+ "pos_x": 0.875,
+ "pos_y": -138.28125,
+ "pos_z": 115.625,
+ "stations": "Pilcher Port,Pritchard Installation,Powell Landing"
+ },
+ {
+ "name": "Rutumukuju",
+ "pos_x": 164.4375,
+ "pos_y": -97.5625,
+ "pos_z": 112,
+ "stations": "Severin's Exile"
+ },
+ {
+ "name": "Ruwachis",
+ "pos_x": 83.96875,
+ "pos_y": -22.3125,
+ "pos_z": 83.125,
+ "stations": "Cseszneky City,Onizuka Gateway,Euclid Gateway,McDaniel Point"
+ },
+ {
+ "name": "Ruwal",
+ "pos_x": 53.75,
+ "pos_y": -96.3125,
+ "pos_z": -130.5,
+ "stations": "Parise Platform,Tito Orbital,Lowry Relay"
+ },
+ {
+ "name": "Ryijin",
+ "pos_x": -65.1875,
+ "pos_y": 66.59375,
+ "pos_z": -30.59375,
+ "stations": "Bryant Gateway,Nemere Depot"
+ },
+ {
+ "name": "Rynchi",
+ "pos_x": 39.375,
+ "pos_y": -218.1875,
+ "pos_z": -64.71875,
+ "stations": "Fan's Exile"
+ },
+ {
+ "name": "Rynchines",
+ "pos_x": 162.84375,
+ "pos_y": 65.03125,
+ "pos_z": 13,
+ "stations": "Nolan Stop,Tavernier Camp"
+ },
+ {
+ "name": "Rynchu",
+ "pos_x": 140.40625,
+ "pos_y": -61.9375,
+ "pos_z": 50.4375,
+ "stations": "van Houten Installation,Finlay-Freundlich Orbital,Dhawan Dock,Vries Landing"
+ },
+ {
+ "name": "Ryu",
+ "pos_x": -47.03125,
+ "pos_y": 83.09375,
+ "pos_z": 22.125,
+ "stations": "Francisco de Almeida Hangar"
+ },
+ {
+ "name": "Ryujin",
+ "pos_x": 82.5,
+ "pos_y": -52.375,
+ "pos_z": 80.34375,
+ "stations": "McIntosh Port,Siegel Survey,Chern's Inheritance"
+ },
+ {
+ "name": "Ryujingit",
+ "pos_x": 18.75,
+ "pos_y": -2.21875,
+ "pos_z": -86.53125,
+ "stations": "Ellis Point,Parmitano Terminal"
+ },
+ {
+ "name": "s Eridani",
+ "pos_x": 57.28125,
+ "pos_y": -115.3125,
+ "pos_z": -15.46875,
+ "stations": "Beg Mines,Tisserand Observatory"
+ },
+ {
+ "name": "Sabi",
+ "pos_x": 174.34375,
+ "pos_y": 32.59375,
+ "pos_z": -22.40625,
+ "stations": "Phillifent Colony"
+ },
+ {
+ "name": "Sabihilo",
+ "pos_x": -48.4375,
+ "pos_y": -76.6875,
+ "pos_z": -39.6875,
+ "stations": "Planck's Claim,Linge Landing,Makarov Orbital"
+ },
+ {
+ "name": "Sabik",
+ "pos_x": -10.59375,
+ "pos_y": 20.46875,
+ "pos_z": 85.28125,
+ "stations": "Swanson Settlement,Stross Vision"
+ },
+ {
+ "name": "Sabikami",
+ "pos_x": -37.75,
+ "pos_y": 102.6875,
+ "pos_z": 82.90625,
+ "stations": "Bowersox Vision"
+ },
+ {
+ "name": "Sabines",
+ "pos_x": 38.59375,
+ "pos_y": -235.1875,
+ "pos_z": 17.03125,
+ "stations": "Woodroffe Terminal,Bartolomeu de Gusmao Vision,Walotsky Enterprise"
+ },
+ {
+ "name": "Sachi Moch",
+ "pos_x": -35.34375,
+ "pos_y": 18.75,
+ "pos_z": 164.84375,
+ "stations": "McQuay Platform,Kandel Lab"
+ },
+ {
+ "name": "Sachmet",
+ "pos_x": 53.5,
+ "pos_y": -4.625,
+ "pos_z": 67,
+ "stations": "Camarda Terminal,Gauss Station,Lindsey Orbital,Hopkins Ring,Comino Port,Hopkins Legacy,Russell Vision"
+ },
+ {
+ "name": "Sachun Dubh",
+ "pos_x": 37.28125,
+ "pos_y": 122.8125,
+ "pos_z": 79.5625,
+ "stations": "Grover Port,Levinson Beacon,Morgan Dock"
+ },
+ {
+ "name": "Sadalachbia",
+ "pos_x": -101.0625,
+ "pos_y": -118.15625,
+ "pos_z": 51.375,
+ "stations": "Kolmogorov Survey,Simak Settlement"
+ },
+ {
+ "name": "Sadhampt",
+ "pos_x": -95.8125,
+ "pos_y": 19.59375,
+ "pos_z": 144.34375,
+ "stations": "Lowry Dock,Aitken Terminal,Back Hub"
+ },
+ {
+ "name": "Sadhant",
+ "pos_x": -69.34375,
+ "pos_y": 71.5625,
+ "pos_z": 31.4375,
+ "stations": "Cameron Hangar,Xing Station"
+ },
+ {
+ "name": "Sadharra",
+ "pos_x": 12.84375,
+ "pos_y": -169.21875,
+ "pos_z": 129.5,
+ "stations": "al-Kashi Beacon"
+ },
+ {
+ "name": "Sadhbh",
+ "pos_x": 61.25,
+ "pos_y": -162.03125,
+ "pos_z": 48.53125,
+ "stations": "Lewis Settlement"
+ },
+ {
+ "name": "Sadhen",
+ "pos_x": -81.03125,
+ "pos_y": -103.875,
+ "pos_z": 17.46875,
+ "stations": "Birkeland Platform,Chorel's Inheritance,Barsanti Port,Garriott Vision,Baudin's Folly"
+ },
+ {
+ "name": "Sadr Region Sector GW-W c1-22",
+ "pos_x": -1792.125,
+ "pos_y": 52.65625,
+ "pos_z": 369.5625,
+ "stations": "Sadr Logistics Depot"
+ },
+ {
+ "name": "Saeliauri",
+ "pos_x": 93.34375,
+ "pos_y": 5.8125,
+ "pos_z": 108.40625,
+ "stations": "Moore Survey,Russell Port,Kennedy Penal colony,Back Relay"
+ },
+ {
+ "name": "Saelina",
+ "pos_x": -60.625,
+ "pos_y": 89.5625,
+ "pos_z": -56.375,
+ "stations": "Lambert's Pride"
+ },
+ {
+ "name": "Saelini",
+ "pos_x": 61.84375,
+ "pos_y": -76.53125,
+ "pos_z": -95.0625,
+ "stations": "Morgan Works"
+ },
+ {
+ "name": "Saelishi",
+ "pos_x": 51.8125,
+ "pos_y": -257.34375,
+ "pos_z": -15,
+ "stations": "Valigursky Prospect,al-Din al-Urdi Mines,Serrao Arsenal"
+ },
+ {
+ "name": "Saffron",
+ "pos_x": 72.875,
+ "pos_y": -117.75,
+ "pos_z": 55.78125,
+ "stations": "Cresswell,Gutierrez Lab,Sleator Port"
+ },
+ {
+ "name": "Saga",
+ "pos_x": 45.625,
+ "pos_y": 6.15625,
+ "pos_z": -133.375,
+ "stations": "Veach Port,Wiley Hub,Pogue Beacon,Illy Hub,Clement Orbital,Zudov Terminal,Savinykh Station,Marshburn Enterprise,Ferguson Enterprise,Chretien Base"
+ },
+ {
+ "name": "Sagalites",
+ "pos_x": 81.53125,
+ "pos_y": 115.0625,
+ "pos_z": 7.625,
+ "stations": "Krusvar Hub,Stross Port,de Caminha Vision,Jones' Progress"
+ },
+ {
+ "name": "Sagar",
+ "pos_x": 54.5625,
+ "pos_y": 92.28125,
+ "pos_z": 80.78125,
+ "stations": "Morgan Market,Muhammad Ibn Battuta Base,Macarthur Port,Glazkov Port,Crouch City"
+ },
+ {
+ "name": "Sagara",
+ "pos_x": 120.0625,
+ "pos_y": 29.34375,
+ "pos_z": -48.65625,
+ "stations": "Coke Settlement"
+ },
+ {
+ "name": "Sagartiaci",
+ "pos_x": 56.5625,
+ "pos_y": -255.03125,
+ "pos_z": -21.15625,
+ "stations": "Kirby Port,Searle Installation,Arago Terminal,Pierres Beacon"
+ },
+ {
+ "name": "Sagartians",
+ "pos_x": -159.25,
+ "pos_y": 65.9375,
+ "pos_z": 59.6875,
+ "stations": "Weierstrass Enterprise,Cook Station,Frobenius Landing,Thompson Survey"
+ },
+ {
+ "name": "Sagi",
+ "pos_x": 3.59375,
+ "pos_y": 140.125,
+ "pos_z": 29.53125,
+ "stations": "Birkeland Hub,Hodgkin Enterprise,Pelliot Base"
+ },
+ {
+ "name": "Saha",
+ "pos_x": 117.78125,
+ "pos_y": -70.78125,
+ "pos_z": 29.40625,
+ "stations": "South Stop"
+ },
+ {
+ "name": "Saha Mati",
+ "pos_x": 7.03125,
+ "pos_y": -57.78125,
+ "pos_z": 98.6875,
+ "stations": "Strekalov Dock,Sinclair Colony"
+ },
+ {
+ "name": "Sahamocoria",
+ "pos_x": 98.25,
+ "pos_y": -164.15625,
+ "pos_z": 167.9375,
+ "stations": "Allen Prospect,Sei Depot,Kuchemann Beacon"
+ },
+ {
+ "name": "Saharish",
+ "pos_x": 78.78125,
+ "pos_y": 61.71875,
+ "pos_z": 102.46875,
+ "stations": "Lysenko Terminal,Olivas City"
+ },
+ {
+ "name": "Saharveti",
+ "pos_x": 157.0625,
+ "pos_y": 15.34375,
+ "pos_z": -0.9375,
+ "stations": "Liouville Legacy"
+ },
+ {
+ "name": "Sahualasta",
+ "pos_x": -72.75,
+ "pos_y": 6.625,
+ "pos_z": 59.09375,
+ "stations": "Kwolek Orbital,den Berg Port,Yang Observatory,Timofeyevich Bastion,Garnier Beacon"
+ },
+ {
+ "name": "Sahun",
+ "pos_x": 139.9375,
+ "pos_y": -80.375,
+ "pos_z": -60.9375,
+ "stations": "Husband Colony,Ahnert Port,Robson Hub"
+ },
+ {
+ "name": "Sairhul",
+ "pos_x": 110.09375,
+ "pos_y": -98.3125,
+ "pos_z": -80.5,
+ "stations": "Kaiser Platform,Oxley Silo"
+ },
+ {
+ "name": "Sairre",
+ "pos_x": -21.21875,
+ "pos_y": 37.375,
+ "pos_z": -82.84375,
+ "stations": "Bentham Hub,Harper Barracks"
+ },
+ {
+ "name": "Sairwar",
+ "pos_x": 18.375,
+ "pos_y": -92.65625,
+ "pos_z": -99.3125,
+ "stations": "Malocello Orbital,Watt-Evans Terminal,Witt Landing,Scott Terminal"
+ },
+ {
+ "name": "Saisiyat",
+ "pos_x": 41.90625,
+ "pos_y": -112.5625,
+ "pos_z": -36.4375,
+ "stations": "Zwicky Hub,Dalgarno Ring,Dubyago Port,Dreyer Terminal,Darboux Base"
+ },
+ {
+ "name": "Sak Chelmir",
+ "pos_x": 55.78125,
+ "pos_y": 40.8125,
+ "pos_z": 19.78125,
+ "stations": "McDonald Colony,Ordway's Progress"
+ },
+ {
+ "name": "Sak Kina",
+ "pos_x": 41.625,
+ "pos_y": 66.75,
+ "pos_z": -61.28125,
+ "stations": "Potagos Settlement"
+ },
+ {
+ "name": "Sak Manka",
+ "pos_x": 43.65625,
+ "pos_y": -105.5,
+ "pos_z": -30.09375,
+ "stations": "Impey Vision,Bonestell Terminal,Linge Vision,Reber Ring,Blish Settlement"
+ },
+ {
+ "name": "Sak Marijem",
+ "pos_x": 100.875,
+ "pos_y": 41.0625,
+ "pos_z": -81.71875,
+ "stations": "Verne Dock,Bordage City"
+ },
+ {
+ "name": "Sak Nicopa",
+ "pos_x": 57.65625,
+ "pos_y": -26.65625,
+ "pos_z": 35.09375,
+ "stations": "Birdseye Platform,Pauli Settlement,Julian Plant"
+ },
+ {
+ "name": "Saka",
+ "pos_x": 125.4375,
+ "pos_y": -43.59375,
+ "pos_z": 56.09375,
+ "stations": "Zel'dovich Station,Bauschinger Station,Mizuno Port"
+ },
+ {
+ "name": "Saka Gaah",
+ "pos_x": 47.46875,
+ "pos_y": -43.75,
+ "pos_z": -53.34375,
+ "stations": "Neumann Horizons,Deere Legacy"
+ },
+ {
+ "name": "Sakaere",
+ "pos_x": -31.03125,
+ "pos_y": -19.96875,
+ "pos_z": 142.71875,
+ "stations": "Kregel Bastion,Bartoe Relay"
+ },
+ {
+ "name": "Sakaja",
+ "pos_x": 152.5,
+ "pos_y": 34.5625,
+ "pos_z": 1.875,
+ "stations": "Staden Orbital,Pangborn's Claim,Fast Vision,Alexandria Terminal,Onnes Installation"
+ },
+ {
+ "name": "Sakampanut",
+ "pos_x": -39.71875,
+ "pos_y": -122.0625,
+ "pos_z": -64.78125,
+ "stations": "Rushworth Enterprise,Ito Gateway,Eyharts Enterprise"
+ },
+ {
+ "name": "Sakar",
+ "pos_x": 145.40625,
+ "pos_y": -32.875,
+ "pos_z": -23.4375,
+ "stations": "Shirley Station,Marlowe's Pride"
+ },
+ {
+ "name": "Sakara",
+ "pos_x": 144.96875,
+ "pos_y": -135.78125,
+ "pos_z": 16.15625,
+ "stations": "Seares Mines,Gehry Hub,Salgari Terminal"
+ },
+ {
+ "name": "Sakarabru",
+ "pos_x": 4.46875,
+ "pos_y": -68.40625,
+ "pos_z": -49.90625,
+ "stations": "Libeskind Terminal,Burbidge's Folly,Hogan Depot,Conway Reach"
+ },
+ {
+ "name": "Sakiana",
+ "pos_x": -112.59375,
+ "pos_y": 103.875,
+ "pos_z": 52.15625,
+ "stations": "Alas Orbital"
+ },
+ {
+ "name": "Sakpata",
+ "pos_x": -9.59375,
+ "pos_y": 51.9375,
+ "pos_z": 56.90625,
+ "stations": "Allen Horizons,Effinger Keep"
+ },
+ {
+ "name": "Sakpatsya",
+ "pos_x": -118.875,
+ "pos_y": -23.21875,
+ "pos_z": 76.375,
+ "stations": "Weyl Outpost,Diophantus Reformatory"
+ },
+ {
+ "name": "Saktas",
+ "pos_x": 31.5,
+ "pos_y": 32.75,
+ "pos_z": -122.375,
+ "stations": "Steinmuller Mines,Mackenzie Mines"
+ },
+ {
+ "name": "Saktassura",
+ "pos_x": -92.65625,
+ "pos_y": -52.5625,
+ "pos_z": -88.875,
+ "stations": "Hoffman Retreat"
+ },
+ {
+ "name": "Sakthau",
+ "pos_x": 127.15625,
+ "pos_y": -64.59375,
+ "pos_z": 75.09375,
+ "stations": "Hagihara Platform"
+ },
+ {
+ "name": "Sakti",
+ "pos_x": -131.5625,
+ "pos_y": -24.21875,
+ "pos_z": -42.8125,
+ "stations": "Andrews Terminal,Young Colony"
+ },
+ {
+ "name": "Saktsak",
+ "pos_x": 1.40625,
+ "pos_y": 16.6875,
+ "pos_z": -12.03125,
+ "stations": "Tarelkin Orbital,Bursch Enterprise,Stephenson Hub,Lind Lab"
+ },
+ {
+ "name": "Sala",
+ "pos_x": 124.375,
+ "pos_y": 15.09375,
+ "pos_z": 26.40625,
+ "stations": "Marshburn Port,Fisher Terminal,Evans Ring,Gantt City,Newton Dock,Hoard Orbital,Jett Station,Onufrienko Installation"
+ },
+ {
+ "name": "Salaallang",
+ "pos_x": 58.875,
+ "pos_y": -175.28125,
+ "pos_z": -62.875,
+ "stations": "Friedrich Peters Gateway,Dassault Gateway,Parkinson Dock,Knorre Legacy"
+ },
+ {
+ "name": "Salan",
+ "pos_x": 65.53125,
+ "pos_y": 55.09375,
+ "pos_z": -121.6875,
+ "stations": "Abasheli Base,Rennie Ring,Davis Orbital,Walker Station,Clark Works"
+ },
+ {
+ "name": "Salangirawi",
+ "pos_x": 16.5625,
+ "pos_y": -115,
+ "pos_z": 43.84375,
+ "stations": "Abetti Station"
+ },
+ {
+ "name": "Salara",
+ "pos_x": -101.65625,
+ "pos_y": 24.375,
+ "pos_z": 40.75,
+ "stations": "Hawley Platform,Glenn Horizons"
+ },
+ {
+ "name": "Salarhul",
+ "pos_x": -120.875,
+ "pos_y": 46.34375,
+ "pos_z": -35.5625,
+ "stations": "Hiraga Gateway,Fibonacci's Progress,Gunn Point"
+ },
+ {
+ "name": "Salava",
+ "pos_x": 119.09375,
+ "pos_y": 115.3125,
+ "pos_z": -6.1875,
+ "stations": "Margulies Colony,Hovell Outpost,Diamond Plant,Krylov's Folly"
+ },
+ {
+ "name": "Sali'naman",
+ "pos_x": -68.59375,
+ "pos_y": -64.71875,
+ "pos_z": 20.3125,
+ "stations": "Russell Ring,Barr Ring"
+ },
+ {
+ "name": "Saliba",
+ "pos_x": 141.53125,
+ "pos_y": 53.5625,
+ "pos_z": -64,
+ "stations": "Vetulani Settlement,Malchiodi Point,Redi Landing"
+ },
+ {
+ "name": "Salibal",
+ "pos_x": 37.25,
+ "pos_y": 39.15625,
+ "pos_z": 45.90625,
+ "stations": "Ostrander Landing,Alpers City,Hiraga Enterprise,RenenBellot Station"
+ },
+ {
+ "name": "Salibala",
+ "pos_x": 53,
+ "pos_y": 19.3125,
+ "pos_z": 123.4375,
+ "stations": "Cooper Orbital,Zindell Barracks"
+ },
+ {
+ "name": "Salibi",
+ "pos_x": 95.71875,
+ "pos_y": -181.21875,
+ "pos_z": 93.28125,
+ "stations": "Clark Dock,Fedden City,Backlund Enterprise,Covington Holdings,Lemonnier Arsenal"
+ },
+ {
+ "name": "Salikians",
+ "pos_x": 157.5625,
+ "pos_y": -0.875,
+ "pos_z": -48.09375,
+ "stations": "Gardner Port,Lambert Base,Poleshchuk Port,Bloomfield Ring"
+ },
+ {
+ "name": "Salimandali",
+ "pos_x": 61.65625,
+ "pos_y": -97.0625,
+ "pos_z": -27.40625,
+ "stations": "Mobius Survey,Kawanishi Enterprise,Klein Plant"
+ },
+ {
+ "name": "Salintaula",
+ "pos_x": 128.0625,
+ "pos_y": 34.78125,
+ "pos_z": 27.90625,
+ "stations": "Potagos Outpost"
+ },
+ {
+ "name": "Salish",
+ "pos_x": 66.53125,
+ "pos_y": 125.53125,
+ "pos_z": 66.65625,
+ "stations": "Szentmartony Mine,Shaver Reach"
+ },
+ {
+ "name": "Salitat",
+ "pos_x": 98.1875,
+ "pos_y": -83.6875,
+ "pos_z": 112.125,
+ "stations": "Anderson Vision,Vyssotsky City"
+ },
+ {
+ "name": "Saliti",
+ "pos_x": -109.09375,
+ "pos_y": 97.8125,
+ "pos_z": -34.65625,
+ "stations": "Holdstock Arena"
+ },
+ {
+ "name": "Sallaecians",
+ "pos_x": 70.5,
+ "pos_y": 33.65625,
+ "pos_z": -49.375,
+ "stations": "Makarov Platform,Reiter Outpost,Harris Works"
+ },
+ {
+ "name": "Salleda",
+ "pos_x": 15.75,
+ "pos_y": -3.5625,
+ "pos_z": 80.8125,
+ "stations": "Gohar Vision,Hobaugh Forum,Casper Terminal"
+ },
+ {
+ "name": "Salledosha",
+ "pos_x": 180.6875,
+ "pos_y": -122.40625,
+ "pos_z": 20.5,
+ "stations": "Tan Outpost"
+ },
+ {
+ "name": "Salliyalar",
+ "pos_x": -22.21875,
+ "pos_y": -142.6875,
+ "pos_z": 79.9375,
+ "stations": "Bereznyak Beacon"
+ },
+ {
+ "name": "Salya",
+ "pos_x": 159.71875,
+ "pos_y": -40.53125,
+ "pos_z": -3.5625,
+ "stations": "Bok Outpost"
+ },
+ {
+ "name": "Sama",
+ "pos_x": -9.71875,
+ "pos_y": -14.34375,
+ "pos_z": 94.625,
+ "stations": "Junlong Platform"
+ },
+ {
+ "name": "Saman",
+ "pos_x": -96.28125,
+ "pos_y": -110.15625,
+ "pos_z": 83.78125,
+ "stations": "Bessemer Platform,Morgan Port"
+ },
+ {
+ "name": "Samar",
+ "pos_x": 71.3125,
+ "pos_y": 68.53125,
+ "pos_z": -142.90625,
+ "stations": "Pournelle Dock"
+ },
+ {
+ "name": "Samas",
+ "pos_x": 55.84375,
+ "pos_y": -173.3125,
+ "pos_z": 166.59375,
+ "stations": "Penzias Relay,Tudela Barracks"
+ },
+ {
+ "name": "Samasaei",
+ "pos_x": 43.6875,
+ "pos_y": -11,
+ "pos_z": -147.125,
+ "stations": "Blackwell Horizons,Chomsky Settlement"
+ },
+ {
+ "name": "Samata",
+ "pos_x": 106.59375,
+ "pos_y": -123.53125,
+ "pos_z": 48.1875,
+ "stations": "Reeves Station,Duque Barracks"
+ },
+ {
+ "name": "Sambaho",
+ "pos_x": -107.46875,
+ "pos_y": 43.90625,
+ "pos_z": -72.75,
+ "stations": "Daley Terminal,Silverberg Retreat"
+ },
+ {
+ "name": "Sambare",
+ "pos_x": -90.3125,
+ "pos_y": -77.1875,
+ "pos_z": -44.84375,
+ "stations": "Guest Dock,Thagard Hub,Drake Base"
+ },
+ {
+ "name": "Samburitja",
+ "pos_x": 90.21875,
+ "pos_y": -161.125,
+ "pos_z": 27.625,
+ "stations": "Abasheli Station,Fraknoi Survey,Tago Prospect,Caidin Landing"
+ },
+ {
+ "name": "Sambuwa",
+ "pos_x": 156.25,
+ "pos_y": -101.65625,
+ "pos_z": 82.65625,
+ "stations": "Lefschetz Keep,Lee Station,Wallis Vision"
+ },
+ {
+ "name": "Samebito",
+ "pos_x": 62.84375,
+ "pos_y": -62.71875,
+ "pos_z": 29.875,
+ "stations": "Vlaicu Settlement"
+ },
+ {
+ "name": "Samen",
+ "pos_x": 68.875,
+ "pos_y": -118.4375,
+ "pos_z": -3.65625,
+ "stations": "Peters Terminal"
+ },
+ {
+ "name": "Sameni",
+ "pos_x": -6.15625,
+ "pos_y": -15.78125,
+ "pos_z": 18.625,
+ "stations": "Tarelkin Landing,Webb Bastion"
+ },
+ {
+ "name": "Samenses",
+ "pos_x": 136.40625,
+ "pos_y": -47.90625,
+ "pos_z": -78.375,
+ "stations": "Sargent Beacon,Wolfe Dock,Griffiths' Folly,Isherwood Base"
+ },
+ {
+ "name": "Samequit",
+ "pos_x": 36.90625,
+ "pos_y": -34.3125,
+ "pos_z": 45.125,
+ "stations": "Braun Enterprise,Whitson Enterprise,Barjavel Installation"
+ },
+ {
+ "name": "Saminicing",
+ "pos_x": 85.125,
+ "pos_y": -145.75,
+ "pos_z": 147.90625,
+ "stations": "Lindblad Port,Arai's Progress,Bharadwaj Landing,Fukushima Platform,Fabricius Holdings"
+ },
+ {
+ "name": "Samkyha",
+ "pos_x": 66.96875,
+ "pos_y": 28.75,
+ "pos_z": -1.21875,
+ "stations": "Rominger Terminal,Abnett Plant"
+ },
+ {
+ "name": "Samnach",
+ "pos_x": 12.875,
+ "pos_y": 15,
+ "pos_z": -139.8125,
+ "stations": "Torricelli Terminal,Cabot Enterprise,Malyshev Stop"
+ },
+ {
+ "name": "Samnienas",
+ "pos_x": -122.875,
+ "pos_y": -73.40625,
+ "pos_z": -11.03125,
+ "stations": "Avicenna Gateway,Mendez Installation,Bernoulli Keep,Otiman City"
+ },
+ {
+ "name": "Samnitics",
+ "pos_x": -6.75,
+ "pos_y": 107.9375,
+ "pos_z": -46,
+ "stations": "Macgregor Dock"
+ },
+ {
+ "name": "Samo",
+ "pos_x": 133.28125,
+ "pos_y": -187.53125,
+ "pos_z": 113.6875,
+ "stations": "Struzan Survey"
+ },
+ {
+ "name": "Samon",
+ "pos_x": -16.9375,
+ "pos_y": -190.53125,
+ "pos_z": 2.9375,
+ "stations": "William Sargent City,Balog Plant,Burnham Dock,Piazzi Orbital,Bharadwaj Keep"
+ },
+ {
+ "name": "Samontalama",
+ "pos_x": -25.78125,
+ "pos_y": -114.8125,
+ "pos_z": 102.4375,
+ "stations": "Burgess Bastion,Arzachel Hangar,Roe Port,Stephan Hangar,Hugh Horizons"
+ },
+ {
+ "name": "Samontia",
+ "pos_x": 30.96875,
+ "pos_y": -191.09375,
+ "pos_z": 130.15625,
+ "stations": "Jewitt Vision,Moore Installation"
+ },
+ {
+ "name": "Samontundji",
+ "pos_x": 19.90625,
+ "pos_y": -57.96875,
+ "pos_z": 158.0625,
+ "stations": "Heng Dock,Linaweaver Installation"
+ },
+ {
+ "name": "San",
+ "pos_x": -96.0625,
+ "pos_y": -24.6875,
+ "pos_z": -175.28125,
+ "stations": "Selous Horizons"
+ },
+ {
+ "name": "San Ahawa",
+ "pos_x": 66.15625,
+ "pos_y": -1.125,
+ "pos_z": 154.46875,
+ "stations": "Cook Landing,Kovalevskaya Settlement"
+ },
+ {
+ "name": "San Camasir",
+ "pos_x": 69.28125,
+ "pos_y": 24.9375,
+ "pos_z": 100.28125,
+ "stations": "Brosnan Terminal,Bates Beacon"
+ },
+ {
+ "name": "San Dan",
+ "pos_x": 129.34375,
+ "pos_y": -135.84375,
+ "pos_z": 152.46875,
+ "stations": "Kurtz Terminal,Denning Dock"
+ },
+ {
+ "name": "San Davokje",
+ "pos_x": 87.59375,
+ "pos_y": -75.21875,
+ "pos_z": -6.0625,
+ "stations": "Fowler City,Gill Station"
+ },
+ {
+ "name": "San Gu",
+ "pos_x": 142.21875,
+ "pos_y": 46.125,
+ "pos_z": 25.78125,
+ "stations": "Neumann Port,Veblen Mines,McArthur's Progress"
+ },
+ {
+ "name": "San Guan",
+ "pos_x": -111.96875,
+ "pos_y": 20.15625,
+ "pos_z": 105.9375,
+ "stations": "Alvarado Port,Stevens Settlement,Fremont Terminal,Sargent Port"
+ },
+ {
+ "name": "San Guazurs",
+ "pos_x": -85.09375,
+ "pos_y": 19.0625,
+ "pos_z": -155.5,
+ "stations": "Mackenzie Relay,Elder Survey,Narbeth Bastion"
+ },
+ {
+ "name": "San Guerets",
+ "pos_x": 57.03125,
+ "pos_y": -146.09375,
+ "pos_z": 94.5,
+ "stations": "Anderson Gateway,Herzog Holdings,Bharadwaj Vision"
+ },
+ {
+ "name": "San Guojin",
+ "pos_x": -101.25,
+ "pos_y": -78.34375,
+ "pos_z": -88.15625,
+ "stations": "Bailey Base,Darboux Plant"
+ },
+ {
+ "name": "San Hsing",
+ "pos_x": 84.21875,
+ "pos_y": -93.75,
+ "pos_z": 103.90625,
+ "stations": "Cartier City"
+ },
+ {
+ "name": "San Huang",
+ "pos_x": 72.5,
+ "pos_y": -17.625,
+ "pos_z": -104.96875,
+ "stations": "Joliot-Curie Port,Phillpotts Laboratory,Sharman Gateway,Boltzmann Vision"
+ },
+ {
+ "name": "San Hun Na",
+ "pos_x": -74.21875,
+ "pos_y": -112.8125,
+ "pos_z": 82.5,
+ "stations": "Whitney City"
+ },
+ {
+ "name": "San Kung",
+ "pos_x": -100.34375,
+ "pos_y": -106,
+ "pos_z": 46.375,
+ "stations": "Cabrera Landing,Soddy Dock,Garay Point"
+ },
+ {
+ "name": "San Lo Wangu",
+ "pos_x": -51.09375,
+ "pos_y": -41.03125,
+ "pos_z": 8.21875,
+ "stations": "Harbaugh Relay"
+ },
+ {
+ "name": "San Mathaa",
+ "pos_x": 44.6875,
+ "pos_y": 94.6875,
+ "pos_z": -46.34375,
+ "stations": "Tapinas Freeport,Slusser Escape,Franklin Enterprise"
+ },
+ {
+ "name": "San Muss",
+ "pos_x": -89.96875,
+ "pos_y": -42.96875,
+ "pos_z": 42.09375,
+ "stations": "Fraas Ring,Gamow Beacon"
+ },
+ {
+ "name": "San Neb Xoc",
+ "pos_x": -76.03125,
+ "pos_y": -123.21875,
+ "pos_z": -11.1875,
+ "stations": "Viktorenko Station,Low Orbital,Flade Dock,Narbeth Plant,Ising Dock,Armstrong Orbital"
+ },
+ {
+ "name": "San Qin Gu",
+ "pos_x": 56.25,
+ "pos_y": -21.65625,
+ "pos_z": -4.40625,
+ "stations": "Wundt Plant,Sinclair Colony,Hopkins Settlement,Kennicott Penal colony"
+ },
+ {
+ "name": "San Saona",
+ "pos_x": 94.15625,
+ "pos_y": -131.28125,
+ "pos_z": 10.21875,
+ "stations": "Evans Hub,i Sola Port"
+ },
+ {
+ "name": "San Ti",
+ "pos_x": -103.1875,
+ "pos_y": -107.21875,
+ "pos_z": 4.625,
+ "stations": "MacCurdy Port"
+ },
+ {
+ "name": "San Tu",
+ "pos_x": 24.96875,
+ "pos_y": 36.8125,
+ "pos_z": -8.78125,
+ "stations": "Chomsky Terminal,Frimout Terminal,Gernhardt Enterprise"
+ },
+ {
+ "name": "San Tzu",
+ "pos_x": 92.4375,
+ "pos_y": 32.375,
+ "pos_z": -94.25,
+ "stations": "Raleigh's Progress"
+ },
+ {
+ "name": "San Wenhot",
+ "pos_x": -21.5625,
+ "pos_y": -40,
+ "pos_z": 89.5,
+ "stations": "Navigator Terminal"
+ },
+ {
+ "name": "San Wu",
+ "pos_x": -3.875,
+ "pos_y": 63.3125,
+ "pos_z": 140.46875,
+ "stations": "Evans Orbital,Anthony de la Roche Dock,Werber Station,Krusvar Port,Brooks Hub"
+ },
+ {
+ "name": "San Wu Gu",
+ "pos_x": 157.125,
+ "pos_y": -131.875,
+ "pos_z": -46.40625,
+ "stations": "Fowler Horizons,Cannon Orbital,VanderMeer Hub,Wang Platform,Smirnova Penal colony,Plucker Plant"
+ },
+ {
+ "name": "San Wuhtire",
+ "pos_x": -62.53125,
+ "pos_y": 41.65625,
+ "pos_z": -138.65625,
+ "stations": "Usachov Survey"
+ },
+ {
+ "name": "San Xiang",
+ "pos_x": 31.78125,
+ "pos_y": -6.125,
+ "pos_z": 120.125,
+ "stations": "Garriott Point,Crown Landing,Leinster Terminal"
+ },
+ {
+ "name": "San Yamurt",
+ "pos_x": 123.8125,
+ "pos_y": -22.625,
+ "pos_z": 110.53125,
+ "stations": "Wood Enterprise,Alcock Reach"
+ },
+ {
+ "name": "San Yax",
+ "pos_x": 57.15625,
+ "pos_y": 14.75,
+ "pos_z": 80,
+ "stations": "Bridger Survey,Baudry Port"
+ },
+ {
+ "name": "San Yulpa",
+ "pos_x": -100.375,
+ "pos_y": 72.6875,
+ "pos_z": -57.84375,
+ "stations": "Klein Legacy,Napier Dock"
+ },
+ {
+ "name": "San Zaihe",
+ "pos_x": 50.0625,
+ "pos_y": -39.3125,
+ "pos_z": 66.3125,
+ "stations": "Ackerman Orbital,Glidden Prospect,Gorbatko Depot"
+ },
+ {
+ "name": "San Zangjel",
+ "pos_x": -103.59375,
+ "pos_y": 115.78125,
+ "pos_z": 71.9375,
+ "stations": "Chandler Dock,Lebesgue Dock,Clarke Dock,Priest Vision,Vinge Keep"
+ },
+ {
+ "name": "Sanai",
+ "pos_x": 38.34375,
+ "pos_y": -113,
+ "pos_z": 42.5,
+ "stations": "Mayer Enterprise,Kowal's Folly,Veron Mines"
+ },
+ {
+ "name": "Sanda",
+ "pos_x": -59.34375,
+ "pos_y": 35.375,
+ "pos_z": -101.03125,
+ "stations": "Waldeck Dock,Sagan Colony,Ledyard Dock,Filter Depot,Pinzon Base"
+ },
+ {
+ "name": "Sandagaray",
+ "pos_x": 43.15625,
+ "pos_y": 48.65625,
+ "pos_z": 66.78125,
+ "stations": "Stirling City"
+ },
+ {
+ "name": "Sandjin",
+ "pos_x": 158.25,
+ "pos_y": -224.875,
+ "pos_z": 74,
+ "stations": "Trimble Relay,Kandrup Mine"
+ },
+ {
+ "name": "Sang Wana",
+ "pos_x": -8.15625,
+ "pos_y": 93.375,
+ "pos_z": -155.40625,
+ "stations": "Bridger Dock,Steele Enterprise"
+ },
+ {
+ "name": "Sangarldwen",
+ "pos_x": 158.65625,
+ "pos_y": -86.71875,
+ "pos_z": 42.125,
+ "stations": "Esposito Orbital,Shipton's Claim"
+ },
+ {
+ "name": "Sangenses",
+ "pos_x": 100.5,
+ "pos_y": -202.34375,
+ "pos_z": 37.40625,
+ "stations": "Suzuki Dock,Rubin Mines,Paola Plant,Paulo da Gama Barracks"
+ },
+ {
+ "name": "Sanggatia",
+ "pos_x": 67.75,
+ "pos_y": -80.21875,
+ "pos_z": -78.21875,
+ "stations": "Vogel Prospect,Frimout Orbital,Dall Installation"
+ },
+ {
+ "name": "Sango",
+ "pos_x": 4.5,
+ "pos_y": 38.75,
+ "pos_z": 51.6875,
+ "stations": "Farrukh Hub,Baxter Station,Lindbohm Port,la Cosa City,Stuart Terminal,Rowley Dock,Krupkat Keep,Sakers Hub,Cavendish Hub,Bessemer Forum"
+ },
+ {
+ "name": "Sanka",
+ "pos_x": 61.0625,
+ "pos_y": -61.65625,
+ "pos_z": 0.875,
+ "stations": "Wilson Depot,Sei Holdings,Alvarez de Pineda Holdings"
+ },
+ {
+ "name": "Sankobuju",
+ "pos_x": 68.375,
+ "pos_y": 95.84375,
+ "pos_z": -61.71875,
+ "stations": "Haisheng Hub"
+ },
+ {
+ "name": "Sankopon",
+ "pos_x": -60.125,
+ "pos_y": -120.4375,
+ "pos_z": -44.75,
+ "stations": "Beaumont Reach,Ozanne's Exile,Tayler Vision"
+ },
+ {
+ "name": "Sankula",
+ "pos_x": -55.59375,
+ "pos_y": 37.8125,
+ "pos_z": -131.03125,
+ "stations": "Bentham Hub,Reis Beacon,Drummond Holdings,Clapperton Platform,Crown Gateway"
+ },
+ {
+ "name": "Sanna",
+ "pos_x": 35.96875,
+ "pos_y": -83.3125,
+ "pos_z": 23.125,
+ "stations": "Ramsbottom Keep,Skyline High,Godfrey's Halt,Adams Reach,Brahe Terminal,Kozeyev's Progress,Piccard Base,Lamarck Dock"
+ },
+ {
+ "name": "Sanoar",
+ "pos_x": -73.90625,
+ "pos_y": 42.9375,
+ "pos_z": -138.5,
+ "stations": "Nylund Horizons,MacLeod Hub,Gaiman Hub,Gallun Depot"
+ },
+ {
+ "name": "Sanopijcha",
+ "pos_x": -80.53125,
+ "pos_y": -130.8125,
+ "pos_z": -52.03125,
+ "stations": "Maire City,Murphy Bastion,Varley's Inheritance,Ron Hubbard Orbital,Clair Mines"
+ },
+ {
+ "name": "Sanos",
+ "pos_x": 73.875,
+ "pos_y": -3.5625,
+ "pos_z": -52.625,
+ "stations": "Crick Enterprise,Brooks Survey,Moorcock Laboratory,Appel Point,Fraley Reach,Apgar Terminal,Allen Ring,Preuss Relay,Bugrov Beacon"
+ },
+ {
+ "name": "Santa Muerte",
+ "pos_x": 94.25,
+ "pos_y": 46.1875,
+ "pos_z": 58.03125,
+ "stations": "Clauss Port"
+ },
+ {
+ "name": "Santal",
+ "pos_x": 66.5625,
+ "pos_y": 13.96875,
+ "pos_z": -23.78125,
+ "stations": "Chorel Legacy"
+ },
+ {
+ "name": "Sante",
+ "pos_x": -59.25,
+ "pos_y": 76.4375,
+ "pos_z": 135.125,
+ "stations": "Appel Point"
+ },
+ {
+ "name": "Santh",
+ "pos_x": 137.375,
+ "pos_y": -105.78125,
+ "pos_z": -53.4375,
+ "stations": "Haro Arena"
+ },
+ {
+ "name": "Santjalan",
+ "pos_x": -27.09375,
+ "pos_y": 32.3125,
+ "pos_z": 106.0625,
+ "stations": "Cantor Terminal,Martinez City,Hausdorff Relay,Tavares City,Dedman Arsenal"
+ },
+ {
+ "name": "Santones",
+ "pos_x": -44.0625,
+ "pos_y": -177.625,
+ "pos_z": 44.15625,
+ "stations": "Wood Mine"
+ },
+ {
+ "name": "Santos Dumont",
+ "pos_x": -9549.53125,
+ "pos_y": -910.34375,
+ "pos_z": 19836.65625,
+ "stations": "Brazilian Dream"
+ },
+ {
+ "name": "Santosageth",
+ "pos_x": -160.34375,
+ "pos_y": -27.28125,
+ "pos_z": -28.53125,
+ "stations": "Jordan's Pride"
+ },
+ {
+ "name": "Santupik",
+ "pos_x": 45.96875,
+ "pos_y": -101.25,
+ "pos_z": 1.0625,
+ "stations": "Helffrich Outpost,Norman Prospect"
+ },
+ {
+ "name": "Santy",
+ "pos_x": 10.5625,
+ "pos_y": -10.53125,
+ "pos_z": 29.75,
+ "stations": "Szilard Terminal,Pauli Penal colony,Noakes City,Collins Oasis"
+ },
+ {
+ "name": "Sanui",
+ "pos_x": 7.8125,
+ "pos_y": -14.625,
+ "pos_z": 159.6875,
+ "stations": "Leclerc Horizons"
+ },
+ {
+ "name": "Sanuku",
+ "pos_x": 20.3125,
+ "pos_y": -27.8125,
+ "pos_z": 5.5,
+ "stations": "Yamazaki Base,Fleming Works"
+ },
+ {
+ "name": "Sanuma",
+ "pos_x": 153.4375,
+ "pos_y": 61.4375,
+ "pos_z": 79.03125,
+ "stations": "Dunyach Gateway,Benyovszky Base"
+ },
+ {
+ "name": "SAO 196806",
+ "pos_x": 87.125,
+ "pos_y": -33.84375,
+ "pos_z": -51.28125,
+ "stations": "Mastracchio Port,England Orbital,Reisman Orbital"
+ },
+ {
+ "name": "SAO 221526",
+ "pos_x": 104.84375,
+ "pos_y": 5.875,
+ "pos_z": 10.875,
+ "stations": "Makarov Station,Burbank Landing,Tarelkin Dock"
+ },
+ {
+ "name": "SAO 82442",
+ "pos_x": 13.4375,
+ "pos_y": 105.375,
+ "pos_z": 4.875,
+ "stations": "Jones Survey,Land Relay,Davis Landing"
+ },
+ {
+ "name": "SAO 92746",
+ "pos_x": -19.125,
+ "pos_y": 92.90625,
+ "pos_z": -37.71875,
+ "stations": "Tilman Prospect,Szentmartony Station,Andree's Pride"
+ },
+ {
+ "name": "SAO 99049",
+ "pos_x": 48.5625,
+ "pos_y": 83,
+ "pos_z": -43.5,
+ "stations": "Schwann Plant"
+ },
+ {
+ "name": "Saon",
+ "pos_x": -101.21875,
+ "pos_y": -44.46875,
+ "pos_z": -70.25,
+ "stations": "Barry Terminal,Rozhdestvensky Survey"
+ },
+ {
+ "name": "Saontiana",
+ "pos_x": -108.5625,
+ "pos_y": -91.125,
+ "pos_z": 19.125,
+ "stations": "Kirtley Platform,Barjavel Port"
+ },
+ {
+ "name": "Saoriosoli",
+ "pos_x": -39.53125,
+ "pos_y": -87.9375,
+ "pos_z": 127.46875,
+ "stations": "Hale Works"
+ },
+ {
+ "name": "Saorsina",
+ "pos_x": -1.875,
+ "pos_y": -190.59375,
+ "pos_z": 95.40625,
+ "stations": "Bok Gateway,Wilder Landing"
+ },
+ {
+ "name": "Sapiety",
+ "pos_x": 75.84375,
+ "pos_y": 69,
+ "pos_z": 114.96875,
+ "stations": "Skripochka Hub,Ito City,Humboldt Station,Liebig Relay"
+ },
+ {
+ "name": "Sapii",
+ "pos_x": 91.0625,
+ "pos_y": -168.59375,
+ "pos_z": 9.90625,
+ "stations": "Svavarsson Point,Hadid Mines,de Kamp Orbital"
+ },
+ {
+ "name": "Sapill",
+ "pos_x": 99.21875,
+ "pos_y": 45.71875,
+ "pos_z": 24.625,
+ "stations": "Leckie Terminal,Coles Ring,Sylvester Terminal,Reamy Relay,Pierres Terminal"
+ },
+ {
+ "name": "Sapites",
+ "pos_x": 80.03125,
+ "pos_y": 56.6875,
+ "pos_z": -28.34375,
+ "stations": "Ingstad Platform,Olsen Hub,Fibonacci Prospect"
+ },
+ {
+ "name": "Sapiticus",
+ "pos_x": 63.53125,
+ "pos_y": -114.125,
+ "pos_z": -66.15625,
+ "stations": "Yakovlev Port"
+ },
+ {
+ "name": "Saptara",
+ "pos_x": -37.90625,
+ "pos_y": 53.625,
+ "pos_z": -165.03125,
+ "stations": "Eskridge Mine"
+ },
+ {
+ "name": "Saptariganu",
+ "pos_x": 43.5,
+ "pos_y": 28.84375,
+ "pos_z": -137.90625,
+ "stations": "Lehtonen Gateway,Lewitt Survey,Tokubei Horizons"
+ },
+ {
+ "name": "Saragir",
+ "pos_x": 25.1875,
+ "pos_y": -160.46875,
+ "pos_z": -84.09375,
+ "stations": "Hertzsprung Terminal,Serre's Claim,Farghani Station"
+ },
+ {
+ "name": "Sarahath",
+ "pos_x": 111.65625,
+ "pos_y": -155.40625,
+ "pos_z": 104.34375,
+ "stations": "McIntosh Market,Rankin Orbital,Forbes Vision,Bode Orbital,Pons Orbital,Wright Orbital,Bingzhen Depot,Shapiro Point"
+ },
+ {
+ "name": "Saraj",
+ "pos_x": -82.28125,
+ "pos_y": 11.375,
+ "pos_z": 32.0625,
+ "stations": "Sturckow Dock"
+ },
+ {
+ "name": "Saramorodia",
+ "pos_x": 52.59375,
+ "pos_y": 76.5,
+ "pos_z": -135.40625,
+ "stations": "Mitchell's Pride,Conklin Depot"
+ },
+ {
+ "name": "Sarana",
+ "pos_x": 91.46875,
+ "pos_y": -14.625,
+ "pos_z": -103.875,
+ "stations": "Blaha Dock,Vaugh Terminal,Debye Survey"
+ },
+ {
+ "name": "Saranggura",
+ "pos_x": 53.1875,
+ "pos_y": -212.09375,
+ "pos_z": -23.28125,
+ "stations": "Dubyago Penal colony"
+ },
+ {
+ "name": "Saranyu",
+ "pos_x": -4.25,
+ "pos_y": -37.15625,
+ "pos_z": -40.59375,
+ "stations": "Gurney Enterprise,McKay Terminal,Hale Survey,Jones Bastion"
+ },
+ {
+ "name": "Saras",
+ "pos_x": 0.125,
+ "pos_y": 60.5,
+ "pos_z": -77.375,
+ "stations": "Pelt Penal colony,Czerny Colony,Dirac Horizons"
+ },
+ {
+ "name": "Saraswati",
+ "pos_x": -9538,
+ "pos_y": -896.40625,
+ "pos_z": 19804.71875,
+ "stations": "Artutanov's Reach"
+ },
+ {
+ "name": "Sarawoyn",
+ "pos_x": 150.3125,
+ "pos_y": -100.5625,
+ "pos_z": 110.375,
+ "stations": "Elson Port"
+ },
+ {
+ "name": "Sardandi",
+ "pos_x": -23.8125,
+ "pos_y": -113.03125,
+ "pos_z": 56.21875,
+ "stations": "Saha Depot,Kennicott Lab"
+ },
+ {
+ "name": "Sardgrivi",
+ "pos_x": -24.78125,
+ "pos_y": -185.28125,
+ "pos_z": -23.65625,
+ "stations": "Dubyago Platform"
+ },
+ {
+ "name": "Saruek",
+ "pos_x": 99.3125,
+ "pos_y": 118.59375,
+ "pos_z": 37.15625,
+ "stations": "Ferguson Port,Ochoa Point"
+ },
+ {
+ "name": "Sarugh",
+ "pos_x": 1.65625,
+ "pos_y": 13.46875,
+ "pos_z": -120,
+ "stations": "Yu Enterprise"
+ },
+ {
+ "name": "Saruna",
+ "pos_x": 161.875,
+ "pos_y": -164.15625,
+ "pos_z": 20.21875,
+ "stations": "Carpenter Depot"
+ },
+ {
+ "name": "Sasan",
+ "pos_x": 140.59375,
+ "pos_y": 84.5625,
+ "pos_z": -19.8125,
+ "stations": "VanderMeer Hub,Quick Gateway"
+ },
+ {
+ "name": "Satero",
+ "pos_x": 106.34375,
+ "pos_y": -92.40625,
+ "pos_z": 104.4375,
+ "stations": "Wagner Installation,Bartini Station,Ortiz Moreno Refinery"
+ },
+ {
+ "name": "Satio",
+ "pos_x": -106.0625,
+ "pos_y": -54.28125,
+ "pos_z": -56.03125,
+ "stations": "Ford Orbital,Block Relay,Abe Base,Lasswitz Depot"
+ },
+ {
+ "name": "Satva",
+ "pos_x": 55.21875,
+ "pos_y": 57.875,
+ "pos_z": 120.90625,
+ "stations": "Borisenko Ring,Pogue Hub,Clervoy Dock,Vercors Depot,Noether Refinery"
+ },
+ {
+ "name": "Satvaneka",
+ "pos_x": 7.65625,
+ "pos_y": 35.5,
+ "pos_z": -115.125,
+ "stations": "Mach City"
+ },
+ {
+ "name": "Satvaramab",
+ "pos_x": 131.90625,
+ "pos_y": -131.15625,
+ "pos_z": -61.59375,
+ "stations": "Roed Odegaard Terminal,Thorne Base"
+ },
+ {
+ "name": "Satvaro",
+ "pos_x": 39.6875,
+ "pos_y": -70.90625,
+ "pos_z": 166.96875,
+ "stations": "Zarnecki's Claim,Dassault Vision"
+ },
+ {
+ "name": "Satvas",
+ "pos_x": 98.15625,
+ "pos_y": 7.3125,
+ "pos_z": -126.875,
+ "stations": "Herreshoff Hangar,Patterson Platform,Lobachevsky Base"
+ },
+ {
+ "name": "Saurami",
+ "pos_x": -123.3125,
+ "pos_y": 99.125,
+ "pos_z": 39.125,
+ "stations": "Rashid Camp"
+ },
+ {
+ "name": "Sauraratec",
+ "pos_x": 120.34375,
+ "pos_y": -97.375,
+ "pos_z": -17.5625,
+ "stations": "Murakami Ring"
+ },
+ {
+ "name": "Saureinja",
+ "pos_x": -82.28125,
+ "pos_y": 56.96875,
+ "pos_z": -83.9375,
+ "stations": "Lichtenberg's Claim,Linge Relay,Maire's Folly"
+ },
+ {
+ "name": "Sauri Ho",
+ "pos_x": 140.71875,
+ "pos_y": -109.65625,
+ "pos_z": -48.71875,
+ "stations": "Karlsefni Asylum,Legendre Mines"
+ },
+ {
+ "name": "Sauris",
+ "pos_x": 62,
+ "pos_y": -228.875,
+ "pos_z": 45.1875,
+ "stations": "Crown Beacon,Trujillo Terminal,Navigator Vision"
+ },
+ {
+ "name": "Saurisan",
+ "pos_x": 15.375,
+ "pos_y": 58.5,
+ "pos_z": -64.90625,
+ "stations": "Parmitano Platform,Samokutyayev Installation,Farrer Landing"
+ },
+ {
+ "name": "Savan",
+ "pos_x": 148.15625,
+ "pos_y": -158.25,
+ "pos_z": 58.8125,
+ "stations": "Airy Dock"
+ },
+ {
+ "name": "Savantzil",
+ "pos_x": -68.21875,
+ "pos_y": -36.53125,
+ "pos_z": -111.75,
+ "stations": "Rice Orbital,He Dock,Peano Base,Ehrenfried Kegel Enterprise"
+ },
+ {
+ "name": "Savaro",
+ "pos_x": 49.9375,
+ "pos_y": 57.59375,
+ "pos_z": 113.46875,
+ "stations": "von Helmont Terminal,Ricardo Depot"
+ },
+ {
+ "name": "Savaroju",
+ "pos_x": 14.84375,
+ "pos_y": 77.09375,
+ "pos_z": 101.96875,
+ "stations": "Khan City,Fung Market,Afanasyev Terminal,Melnick Terminal,Pontes Port,Mieville Beacon"
+ },
+ {
+ "name": "Savas",
+ "pos_x": 74.03125,
+ "pos_y": 7.875,
+ "pos_z": 147.53125,
+ "stations": "Cartier Penal colony,Lee Vision,Cardano's Progress,Ahmed Orbital"
+ },
+ {
+ "name": "Savi",
+ "pos_x": -82.78125,
+ "pos_y": 64.4375,
+ "pos_z": -90.21875,
+ "stations": "Hammond Gateway,Vela Hub,Glidden Gateway,Grego Depot,Wheeler Terminal,Dalton Terminal,Gemar City,Farkas Hub,Burstein Horizons,Herreshoff Refinery"
+ },
+ {
+ "name": "Savian",
+ "pos_x": -27.5,
+ "pos_y": 21.125,
+ "pos_z": -146.34375,
+ "stations": "Bierce Prospect,Hardwick Installation,Ericsson Vision"
+ },
+ {
+ "name": "Savii",
+ "pos_x": 35.1875,
+ "pos_y": -33.4375,
+ "pos_z": 67.28125,
+ "stations": "Parise Point"
+ },
+ {
+ "name": "Savincates",
+ "pos_x": 57,
+ "pos_y": 158.90625,
+ "pos_z": -40.34375,
+ "stations": "Bruce Settlement,Hardy Colony,Resnick Vision"
+ },
+ {
+ "name": "Savir",
+ "pos_x": -50.5625,
+ "pos_y": 135.15625,
+ "pos_z": 87.46875,
+ "stations": "Christian Escape"
+ },
+ {
+ "name": "Saviriku",
+ "pos_x": 25.59375,
+ "pos_y": 30.4375,
+ "pos_z": 156.78125,
+ "stations": "von Bellingshausen's Progress,Artin's Folly"
+ },
+ {
+ "name": "Sawadbhakui",
+ "pos_x": -75.3125,
+ "pos_y": 104.34375,
+ "pos_z": -127.09375,
+ "stations": "Lynn Dock,Stiegler Landing"
+ },
+ {
+ "name": "Sawahinn",
+ "pos_x": -165.6875,
+ "pos_y": 0.78125,
+ "pos_z": -52.5,
+ "stations": "Cardano Hub,Bushkov Depot,Manarov Enterprise,Lundwall's Folly,Efremov Beacon,Citroen Enterprise"
+ },
+ {
+ "name": "Sawait",
+ "pos_x": 156.375,
+ "pos_y": -98.25,
+ "pos_z": -31.125,
+ "stations": "Bainbridge Terminal,Suzuki Station,Carpenter Colony,Jones Colony,Dickson Holdings"
+ },
+ {
+ "name": "Sawali",
+ "pos_x": 97.90625,
+ "pos_y": 23.15625,
+ "pos_z": -52.25,
+ "stations": "Aksyonov Landing,Liebig Landing,Swanson Colony"
+ },
+ {
+ "name": "Sawar",
+ "pos_x": 95.40625,
+ "pos_y": -223.625,
+ "pos_z": 40.90625,
+ "stations": "Payne-Gaposchkin Mines,Gould Terminal,Norman Holdings,Kulin Base"
+ },
+ {
+ "name": "Sawasir",
+ "pos_x": 44.875,
+ "pos_y": 162.5,
+ "pos_z": 24.625,
+ "stations": "Bohnhoff Base,Tsunenaga Outpost"
+ },
+ {
+ "name": "Saya",
+ "pos_x": 62.5625,
+ "pos_y": 42.96875,
+ "pos_z": 149.21875,
+ "stations": "Dzhanibekov Station,Hale Point"
+ },
+ {
+ "name": "Sayango",
+ "pos_x": 37.15625,
+ "pos_y": -37.15625,
+ "pos_z": 70.46875,
+ "stations": "Seamans Survey"
+ },
+ {
+ "name": "Sceptrum",
+ "pos_x": 46.78125,
+ "pos_y": -63.5625,
+ "pos_z": -76.4375,
+ "stations": "Clifton Orbital,Benyovszky Station,Altshuller Gateway,Norgay City,Heceta Orbital,Hannu Settlement,Curbeam Point,Fossum Colony"
+ },
+ {
+ "name": "Scirians",
+ "pos_x": 178.75,
+ "pos_y": -164.59375,
+ "pos_z": 94,
+ "stations": "Kawasato Port"
+ },
+ {
+ "name": "Scirtari",
+ "pos_x": 68.6875,
+ "pos_y": -231.625,
+ "pos_z": 78.3125,
+ "stations": "Herzog Port,Fieseler Hub,Garnier Installation"
+ },
+ {
+ "name": "Scirth",
+ "pos_x": 34.875,
+ "pos_y": -98.15625,
+ "pos_z": -69.59375,
+ "stations": "Gabriel City,Bordage Hub,Jenkinson Terminal,Remec City,Webb Orbital,Pratchett Installation,Herjulfsson Keep,Krusvar Dock,Powers Vista,Hopkinson Terminal,Lichtenberg City,Nemere Keep,Drake Relay,Margulies Station,Urkovic Gateway"
+ },
+ {
+ "name": "Scirtoi",
+ "pos_x": -14.8125,
+ "pos_y": -223.96875,
+ "pos_z": 11.78125,
+ "stations": "Tago Dock,Roche Colony"
+ },
+ {
+ "name": "Scirtoq",
+ "pos_x": 35.125,
+ "pos_y": -202.3125,
+ "pos_z": 81.375,
+ "stations": "Shapiro Prospect,Deslandres Station,Rutan Terminal"
+ },
+ {
+ "name": "Scordisci",
+ "pos_x": -39.71875,
+ "pos_y": -91.65625,
+ "pos_z": 12.125,
+ "stations": "Chomsky Ring,Hawley Station"
+ },
+ {
+ "name": "Scori",
+ "pos_x": 18.40625,
+ "pos_y": -18.375,
+ "pos_z": 23.3125,
+ "stations": "Napier Relay,Lonchakov Gateway,Pascal Survey"
+ },
+ {
+ "name": "Scories",
+ "pos_x": 81.65625,
+ "pos_y": -52.125,
+ "pos_z": -153.46875,
+ "stations": "Fanning Outpost,Belyanin Works,Wolfe Terminal"
+ },
+ {
+ "name": "Scoriones",
+ "pos_x": 124.875,
+ "pos_y": -133.0625,
+ "pos_z": -49.6875,
+ "stations": "Armero Survey"
+ },
+ {
+ "name": "Scorix",
+ "pos_x": 110.8125,
+ "pos_y": -79.1875,
+ "pos_z": -62.15625,
+ "stations": "Abnett Beacon,Busemann Mines,Wild Settlement"
+ },
+ {
+ "name": "Scorpii Sector CL-X b1-2",
+ "pos_x": 64.5,
+ "pos_y": 35.96875,
+ "pos_z": 104.25,
+ "stations": "Grabe Holdings,Reynolds Horizons"
+ },
+ {
+ "name": "Scorpii Sector CL-Y d100",
+ "pos_x": 6.21875,
+ "pos_y": 28.15625,
+ "pos_z": 161.84375,
+ "stations": "Cavendish Keep"
+ },
+ {
+ "name": "Scorpii Sector CQ-Y c22",
+ "pos_x": 82.90625,
+ "pos_y": 32.59375,
+ "pos_z": 104.40625,
+ "stations": "Schroeder's Folly,Bunnell's Inheritance"
+ },
+ {
+ "name": "Scorpii Sector CQ-Y c24",
+ "pos_x": 64,
+ "pos_y": 33.71875,
+ "pos_z": 113.75,
+ "stations": "Nikolayev Terminal,McCaffrey Landing"
+ },
+ {
+ "name": "Scorpii Sector DB-X b1-8",
+ "pos_x": -2,
+ "pos_y": -1.46875,
+ "pos_z": 99.6875,
+ "stations": "The Iron Claw"
+ },
+ {
+ "name": "Scorpii Sector DL-Y d129",
+ "pos_x": 48.6875,
+ "pos_y": -21.34375,
+ "pos_z": 137.34375,
+ "stations": "Loncke Landing,Clayton Survey"
+ },
+ {
+ "name": "Scorpii Sector DL-Y d157",
+ "pos_x": 75.28125,
+ "pos_y": 24.84375,
+ "pos_z": 156.09375,
+ "stations": "Remek's Inheritance"
+ },
+ {
+ "name": "Scorpii Sector EL-Y c29",
+ "pos_x": 63.375,
+ "pos_y": 3.65625,
+ "pos_z": 130.625,
+ "stations": "Dukaj Prospect,Pribylov Base"
+ },
+ {
+ "name": "Scorpii Sector ER-V b2-5",
+ "pos_x": 20.59375,
+ "pos_y": 47.6875,
+ "pos_z": 119.53125,
+ "stations": "Cadamosto Lab,Webb Depot"
+ },
+ {
+ "name": "Scorpii Sector EW-W c1-27",
+ "pos_x": 5.375,
+ "pos_y": 18.71875,
+ "pos_z": 146.625,
+ "stations": "Manning Relay,Reis Holdings,Serling Base"
+ },
+ {
+ "name": "Scorpii Sector FM-V b2-5",
+ "pos_x": 5.46875,
+ "pos_y": 32.53125,
+ "pos_z": 118.1875,
+ "stations": "Astur's Vault"
+ },
+ {
+ "name": "Scorpii Sector FW-W c1-14",
+ "pos_x": 21.78125,
+ "pos_y": 52,
+ "pos_z": 139.65625,
+ "stations": "Wingqvist Prospect"
+ },
+ {
+ "name": "Scorpii Sector FW-W c1-15",
+ "pos_x": 42.25,
+ "pos_y": 23.75,
+ "pos_z": 140.875,
+ "stations": "Stevens Town"
+ },
+ {
+ "name": "Scorpii Sector FW-W c1-20",
+ "pos_x": 34.34375,
+ "pos_y": 27.46875,
+ "pos_z": 172.4375,
+ "stations": "Becquerel Settlement"
+ },
+ {
+ "name": "Scorpii Sector FW-W c1-29",
+ "pos_x": 16.15625,
+ "pos_y": 22.875,
+ "pos_z": 138.03125,
+ "stations": "Bischoff Prospect,Baker Point,Mitchell Escape"
+ },
+ {
+ "name": "Scorpii Sector FW-W c1-7",
+ "pos_x": 32.34375,
+ "pos_y": 23.15625,
+ "pos_z": 138.40625,
+ "stations": "Tanner's Folly,Siegel Relay"
+ },
+ {
+ "name": "Scorpii Sector GR-V b2-1",
+ "pos_x": 55.46875,
+ "pos_y": 38.34375,
+ "pos_z": 123.125,
+ "stations": "Shirley Enterprise,Collins Holdings"
+ },
+ {
+ "name": "Scorpii Sector GR-W c1-12",
+ "pos_x": -18.71875,
+ "pos_y": 10,
+ "pos_z": 135.09375,
+ "stations": "Reed Beacon,Santarem Base,Bethke Plant"
+ },
+ {
+ "name": "Scorpii Sector GR-W c1-29",
+ "pos_x": -13.5625,
+ "pos_y": 13.96875,
+ "pos_z": 136.375,
+ "stations": "Tavares Mine,Nobel Depot,Pinto Hub"
+ },
+ {
+ "name": "Scorpii Sector HR-W c1-19",
+ "pos_x": 30.84375,
+ "pos_y": -9.875,
+ "pos_z": 174.5,
+ "stations": "Spielberg's Progress,Sturt Works"
+ },
+ {
+ "name": "Scorpii Sector HR-W c1-21",
+ "pos_x": 24.28125,
+ "pos_y": 2.9375,
+ "pos_z": 146.28125,
+ "stations": "Searfoss Depot"
+ },
+ {
+ "name": "Scorpii Sector HR-W c1-25",
+ "pos_x": 18.65625,
+ "pos_y": 0.5625,
+ "pos_z": 172.40625,
+ "stations": "Frobenius' Progress"
+ },
+ {
+ "name": "Scorpii Sector HR-W c1-34",
+ "pos_x": 18.40625,
+ "pos_y": 11.34375,
+ "pos_z": 163.21875,
+ "stations": "Dobrovolskiy Terminal"
+ },
+ {
+ "name": "Scorpii Sector IR-W c1-22",
+ "pos_x": 76.15625,
+ "pos_y": 3.46875,
+ "pos_z": 163.125,
+ "stations": "Cochrane's Inheritance,Pratchett Enterprise"
+ },
+ {
+ "name": "Scorpii Sector JM-W c1-14",
+ "pos_x": 24.25,
+ "pos_y": -30.90625,
+ "pos_z": 150.28125,
+ "stations": "Kovalyonok Works,Lockhart's Folly"
+ },
+ {
+ "name": "Scorpii Sector JW-W b1-3",
+ "pos_x": 79.125,
+ "pos_y": -6.125,
+ "pos_z": 107.59375,
+ "stations": "Coulomb Relay"
+ },
+ {
+ "name": "Scorpii Sector KH-V b2-3",
+ "pos_x": 68.03125,
+ "pos_y": 14.09375,
+ "pos_z": 128.125,
+ "stations": "Lewis' Inheritance,Tolstoi Horizons"
+ },
+ {
+ "name": "Scorpii Sector KM-W c1-5",
+ "pos_x": 63,
+ "pos_y": -32.9375,
+ "pos_z": 143,
+ "stations": "Drake Landing,Morrison Beacon"
+ },
+ {
+ "name": "Scorpii Sector KS-T b3-3",
+ "pos_x": 19.5,
+ "pos_y": 28.96875,
+ "pos_z": 139.75,
+ "stations": "Liebig Lab,Griffiths Keep"
+ },
+ {
+ "name": "Scorpii Sector KX-T b3-6",
+ "pos_x": 73.21875,
+ "pos_y": 43.09375,
+ "pos_z": 146.65625,
+ "stations": "Atwood Settlement,Bolger Enterprise"
+ },
+ {
+ "name": "Scorpii Sector NS-T b3-3",
+ "pos_x": 88.09375,
+ "pos_y": 18.5,
+ "pos_z": 151.15625,
+ "stations": "Vespucci Depot"
+ },
+ {
+ "name": "Scorpii Sector OI-T b3-4",
+ "pos_x": 25.65625,
+ "pos_y": -19.28125,
+ "pos_z": 148.6875,
+ "stations": "Pirsan Installation"
+ },
+ {
+ "name": "Scorpii Sector ON-T b3-6",
+ "pos_x": 69.9375,
+ "pos_y": -1.46875,
+ "pos_z": 152.3125,
+ "stations": "Onnes Horizons"
+ },
+ {
+ "name": "Scorpii Sector PI-T b3-1",
+ "pos_x": 37.3125,
+ "pos_y": -6.53125,
+ "pos_z": 154.375,
+ "stations": "Adams' Inheritance"
+ },
+ {
+ "name": "Scorpii Sector PI-T b3-2",
+ "pos_x": 45.5,
+ "pos_y": -8.40625,
+ "pos_z": 150.09375,
+ "stations": "Smith Reach"
+ },
+ {
+ "name": "Scotaya",
+ "pos_x": 14.1875,
+ "pos_y": 42.25,
+ "pos_z": 75.65625,
+ "stations": "Laplace Enterprise,Flade Platform"
+ },
+ {
+ "name": "Scottii",
+ "pos_x": 124,
+ "pos_y": -116.40625,
+ "pos_z": -31.65625,
+ "stations": "Babakin Dock"
+ },
+ {
+ "name": "Scylla",
+ "pos_x": 54.9375,
+ "pos_y": -50.4375,
+ "pos_z": -34.28125,
+ "stations": "Edison Gateway,Qureshi Port,Rzeppa City,Carrier City,Makarov Orbital,Boe Dock,Ham Dock,Fadlan Gateway,Tesla Port,Bates' Pride,Komarov Point,Nagel City"
+ },
+ {
+ "name": "Scythia",
+ "pos_x": 6.75,
+ "pos_y": -76.90625,
+ "pos_z": -150.34375,
+ "stations": "Tayler Prospect"
+ },
+ {
+ "name": "Scythians",
+ "pos_x": 35.0625,
+ "pos_y": -81.8125,
+ "pos_z": 142.96875,
+ "stations": "Giancola Vision,Horrocks Camp,Valz Relay"
+ },
+ {
+ "name": "Scythimichs",
+ "pos_x": -127.125,
+ "pos_y": -14.71875,
+ "pos_z": 105.6875,
+ "stations": "Braun Landing,Wilson Settlement"
+ },
+ {
+ "name": "Scythipik",
+ "pos_x": -89.34375,
+ "pos_y": -68.1875,
+ "pos_z": 30.8125,
+ "stations": "Sacco Base,Pettit Prospect"
+ },
+ {
+ "name": "Scythodio",
+ "pos_x": -86.71875,
+ "pos_y": -41.59375,
+ "pos_z": 39.0625,
+ "stations": "Lichtenberg Station"
+ },
+ {
+ "name": "Seagull Sector DL-Y d3",
+ "pos_x": 2608.15625,
+ "pos_y": -181.96875,
+ "pos_z": -2692.28125,
+ "stations": "Hell Port"
+ },
+ {
+ "name": "Sebeleni",
+ "pos_x": -28.40625,
+ "pos_y": -39.25,
+ "pos_z": -150.34375,
+ "stations": "Fraas Hangar"
+ },
+ {
+ "name": "Secomi",
+ "pos_x": 155.96875,
+ "pos_y": -0.375,
+ "pos_z": -39.3125,
+ "stations": "Hackworth Ring"
+ },
+ {
+ "name": "Secoya",
+ "pos_x": 64.3125,
+ "pos_y": -63.625,
+ "pos_z": 62.375,
+ "stations": "Joy Port,Resnik Hub,Common Reach,Pond Port,Jolliet's Claim,Cottenot Legacy,Nagata Hub,Suydam Hub,Ulloa Port,Moore Mines,Houten-Groeneveld Port"
+ },
+ {
+ "name": "Secoyans",
+ "pos_x": 129.1875,
+ "pos_y": 47.375,
+ "pos_z": 54.625,
+ "stations": "Volynov Vision,Marshall City,Dedman Survey,J. G. Ballard Barracks"
+ },
+ {
+ "name": "Sedi",
+ "pos_x": 46.03125,
+ "pos_y": 94.28125,
+ "pos_z": -37.34375,
+ "stations": "Feustel Orbital,Beregovoi Orbital,Fujimori Dock,Carlisle Installation"
+ },
+ {
+ "name": "Sedia",
+ "pos_x": -2.75,
+ "pos_y": -6.1875,
+ "pos_z": 98.375,
+ "stations": "Collins Horizons,Covey Settlement,Mayer Vision"
+ },
+ {
+ "name": "Sedimo",
+ "pos_x": -108.46875,
+ "pos_y": 109.34375,
+ "pos_z": 57.3125,
+ "stations": "Hume Beacon,Tarentum Terminal,Zindell Landing,Samos Platform,Carr Dock"
+ },
+ {
+ "name": "Seditya",
+ "pos_x": -96.125,
+ "pos_y": 21.0625,
+ "pos_z": 46.65625,
+ "stations": "Gibson Survey,Zamyatin Landing,Serre Prospect"
+ },
+ {
+ "name": "Sedna",
+ "pos_x": 94.21875,
+ "pos_y": 2.03125,
+ "pos_z": -12.3125,
+ "stations": "Brooks Hold,Herreshoff Beacon,Dampier Enterprise"
+ },
+ {
+ "name": "Sedu",
+ "pos_x": 123.5,
+ "pos_y": -148.40625,
+ "pos_z": -23.25,
+ "stations": "Gill Enterprise,Richer Vision,Schilling Base"
+ },
+ {
+ "name": "Seedi",
+ "pos_x": 30.46875,
+ "pos_y": 140.25,
+ "pos_z": -38.0625,
+ "stations": "Westerfeld Refinery,Shaw Settlement"
+ },
+ {
+ "name": "Seediansi",
+ "pos_x": -15.59375,
+ "pos_y": -28.9375,
+ "pos_z": -6.5,
+ "stations": "Grover Orbital"
+ },
+ {
+ "name": "Seelet",
+ "pos_x": -62.15625,
+ "pos_y": 57.375,
+ "pos_z": 24.84375,
+ "stations": "Alas Orbital,Midgeley Orbital,Searfoss Dock,Tokubei Bastion,Alexander Settlement,Dedman Horizons"
+ },
+ {
+ "name": "Sefrys",
+ "pos_x": -55.75,
+ "pos_y": -53,
+ "pos_z": 161.125,
+ "stations": "Mercenary's Respite"
+ },
+ {
+ "name": "Segais",
+ "pos_x": 12.625,
+ "pos_y": -28.46875,
+ "pos_z": 18.53125,
+ "stations": "Bates Orbital,Fearn Terminal,Salgari Station,Palmer Horizons"
+ },
+ {
+ "name": "Segeirre",
+ "pos_x": 85.53125,
+ "pos_y": -15.125,
+ "pos_z": -91.5,
+ "stations": "Rashid Beacon,Bosch Beacon,Murray Barracks"
+ },
+ {
+ "name": "Segestani",
+ "pos_x": 28.71875,
+ "pos_y": 115,
+ "pos_z": 111.65625,
+ "stations": "Clark Dock,Mawson Point,Marusek Dock,Sakers Laboratory"
+ },
+ {
+ "name": "Segezitis",
+ "pos_x": 174.53125,
+ "pos_y": -68.21875,
+ "pos_z": -26.90625,
+ "stations": "Gorey Depot,McKee Landing"
+ },
+ {
+ "name": "Seginus",
+ "pos_x": -32.53125,
+ "pos_y": 79.1875,
+ "pos_z": 14.1875,
+ "stations": "Taylor Ring,Jones Station,Soukup Hub,Meyrink Port,Sagan Dock,Pangborn Holdings,Niven City,McDaniel Beacon,Morris Orbital,Siodmak Exchange,Bokeili Observatory,Santarem Relay,Mendez Prospect,al-Haytham Orbital"
+ },
+ {
+ "name": "Segnen",
+ "pos_x": 179.25,
+ "pos_y": -161.0625,
+ "pos_z": 23.0625,
+ "stations": "Lee Depot,Jael Works"
+ },
+ {
+ "name": "Segnir",
+ "pos_x": 118.5625,
+ "pos_y": -119.875,
+ "pos_z": 115.15625,
+ "stations": "Condit Platform,Janjetov Base,Xuesen Horizons"
+ },
+ {
+ "name": "Sego",
+ "pos_x": -134.21875,
+ "pos_y": -30.96875,
+ "pos_z": -102.5,
+ "stations": "Steiner Reach"
+ },
+ {
+ "name": "Segobo",
+ "pos_x": -97.5,
+ "pos_y": -34.34375,
+ "pos_z": 19.25,
+ "stations": "Weil Penal colony,Pratchett Platform,Nobel Outpost"
+ },
+ {
+ "name": "Segon",
+ "pos_x": -48.96875,
+ "pos_y": -0.6875,
+ "pos_z": -96,
+ "stations": "O'Leary Vision,Russ Landing,Dirichlet Laboratory,Kube-McDowell's Folly,Carrasco Orbital"
+ },
+ {
+ "name": "Segontiaci",
+ "pos_x": 131.65625,
+ "pos_y": -122.78125,
+ "pos_z": 3,
+ "stations": "Babcock Mines,Youll Vision,Cooper Enterprise,Roe Dock"
+ },
+ {
+ "name": "Segou",
+ "pos_x": -113.25,
+ "pos_y": 23.90625,
+ "pos_z": 59.96875,
+ "stations": "Merril Mine"
+ },
+ {
+ "name": "Segovan",
+ "pos_x": -105.8125,
+ "pos_y": -18.34375,
+ "pos_z": -127.5625,
+ "stations": "Ramsbottom Hub,Smith Terminal,Bramah Market,Hertz Enterprise"
+ },
+ {
+ "name": "Segovas",
+ "pos_x": 165.59375,
+ "pos_y": -134.5,
+ "pos_z": -41.3125,
+ "stations": "Yuzhe Dock,Thollon Works,Dahm Works"
+ },
+ {
+ "name": "Segoveduwa",
+ "pos_x": 71.34375,
+ "pos_y": -94.8125,
+ "pos_z": 48.59375,
+ "stations": "Mason Hub,Dolgov Port,Lalande Station,Nojiri Refinery"
+ },
+ {
+ "name": "Segovit",
+ "pos_x": 8.65625,
+ "pos_y": -57.84375,
+ "pos_z": 19.8125,
+ "stations": "Pauling Settlement,Parise Colony,Hiraga Relay"
+ },
+ {
+ "name": "Seguaje",
+ "pos_x": -4.9375,
+ "pos_y": -24.5,
+ "pos_z": -161.3125,
+ "stations": "Agassiz Station,Chilton City,Lucretius Station,Salgari Enterprise,Artin Keep"
+ },
+ {
+ "name": "Sehali",
+ "pos_x": 26.75,
+ "pos_y": 132.5625,
+ "pos_z": -49.96875,
+ "stations": "Krupkat Terminal,Everest Station,Froude Terminal,Mieville Hub"
+ },
+ {
+ "name": "Seharis",
+ "pos_x": 91.96875,
+ "pos_y": -65.40625,
+ "pos_z": 17.96875,
+ "stations": "Luu Works"
+ },
+ {
+ "name": "Sekenks",
+ "pos_x": -121.78125,
+ "pos_y": 76.90625,
+ "pos_z": -101.5625,
+ "stations": "Russell Dock,Disch Dock,Zalyotin Holdings,Sagan's Progress"
+ },
+ {
+ "name": "Seket",
+ "pos_x": 47.78125,
+ "pos_y": -123.03125,
+ "pos_z": -59.96875,
+ "stations": "Zubrin Mines,Riazuddin Settlement"
+ },
+ {
+ "name": "Sekh",
+ "pos_x": 124.96875,
+ "pos_y": -122.90625,
+ "pos_z": 28.8125,
+ "stations": "Abel Market,Beer Vision,Bailey Holdings,Moffitt Enterprise,Nixon Depot"
+ },
+ {
+ "name": "Sekhemet",
+ "pos_x": 33.1875,
+ "pos_y": 30.375,
+ "pos_z": -29.21875,
+ "stations": "Brunton Hub,Nasmyth Station,Vittori Gateway"
+ },
+ {
+ "name": "Sekhenses",
+ "pos_x": -12.90625,
+ "pos_y": -19.09375,
+ "pos_z": 27.625,
+ "stations": "Herzfeld Hub,Carver Station,Lindsey Depot,Werner von Siemens Settlement"
+ },
+ {
+ "name": "Sekhereke",
+ "pos_x": 137.0625,
+ "pos_y": -33.59375,
+ "pos_z": 49.1875,
+ "stations": "Chebyshev Base,Delaunay Gateway,Anderson Station,Abetti Station"
+ },
+ {
+ "name": "Sekhmetures",
+ "pos_x": -149.03125,
+ "pos_y": -23.125,
+ "pos_z": 10.15625,
+ "stations": "Forfait Ring,Lambert Horizons,d'Eyncourt Observatory,Williams Horizons"
+ },
+ {
+ "name": "Selebegua",
+ "pos_x": 58.75,
+ "pos_y": 61.125,
+ "pos_z": 25.59375,
+ "stations": "Higginbotham City,Crown Dock,Meitner Hub,Bykovsky Gateway,Baird Orbital,Cenker Enterprise,Sarafanov Dock"
+ },
+ {
+ "name": "Seleru Bao",
+ "pos_x": -13.9375,
+ "pos_y": -62.96875,
+ "pos_z": -70.03125,
+ "stations": "Leinster Ring,Laing Ring,Hasse Horizons"
+ },
+ {
+ "name": "Selgalam",
+ "pos_x": 136,
+ "pos_y": -26.15625,
+ "pos_z": 90.25,
+ "stations": "Levi-Montalcini Dock"
+ },
+ {
+ "name": "Selgeth",
+ "pos_x": -44.1875,
+ "pos_y": 37.1875,
+ "pos_z": -115.34375,
+ "stations": "Back Orbital,Raleigh Base,Pohl Depot,Moriarty Platform"
+ },
+ {
+ "name": "Seliacha",
+ "pos_x": -21.9375,
+ "pos_y": -64.875,
+ "pos_z": 58.25,
+ "stations": "Selye Plant,Lee Base,Runco Orbital"
+ },
+ {
+ "name": "Selianciens",
+ "pos_x": -54.8125,
+ "pos_y": -22.6875,
+ "pos_z": 90.75,
+ "stations": "Jones Station,Wilson Port,Krusvar Enterprise,Alexeyev Dock"
+ },
+ {
+ "name": "Selinja",
+ "pos_x": -65.40625,
+ "pos_y": 132.9375,
+ "pos_z": 85.28125,
+ "stations": "Thurston Depot"
+ },
+ {
+ "name": "Selisao Jiu",
+ "pos_x": 46,
+ "pos_y": -210.21875,
+ "pos_z": -7.71875,
+ "stations": "Urkovic Holdings,Haack Survey,Acharya's Progress"
+ },
+ {
+ "name": "Seliu Benas",
+ "pos_x": 130.8125,
+ "pos_y": -208.8125,
+ "pos_z": -95.9375,
+ "stations": "Tan Orbital,Speke Hub,Flagg Terminal"
+ },
+ {
+ "name": "Selk'nam",
+ "pos_x": -30.6875,
+ "pos_y": 107.375,
+ "pos_z": -81.78125,
+ "stations": "Soddy City,Ayerdhal's Folly,Precourt Hub,Selye Orbital,Ride Depot"
+ },
+ {
+ "name": "Selkabere",
+ "pos_x": 126.78125,
+ "pos_y": -16.1875,
+ "pos_z": 127.875,
+ "stations": "Regiomontanus Colony,Bradshaw Horizons,Levi-Civita Enterprise,Marlowe Platform"
+ },
+ {
+ "name": "Selkadiae",
+ "pos_x": 172.09375,
+ "pos_y": -180.625,
+ "pos_z": 86.15625,
+ "stations": "de Kamp Dock,Payne-Gaposchkin Horizons,Scotti Orbital"
+ },
+ {
+ "name": "Selkana",
+ "pos_x": 64.59375,
+ "pos_y": 74.125,
+ "pos_z": 87.46875,
+ "stations": "Pythagoras Port,Lawrence Port,Hoffman Market,Serebrov Dock,Walker Port,Bolotov Enterprise,Gaffney Enterprise,Resnick Enterprise,Herbert Hub"
+ },
+ {
+ "name": "Selkande",
+ "pos_x": 82.53125,
+ "pos_y": -102.34375,
+ "pos_z": 72.65625,
+ "stations": "Lewis Gateway,Lehtonen Terminal"
+ },
+ {
+ "name": "Selkit",
+ "pos_x": 118.9375,
+ "pos_y": -240.9375,
+ "pos_z": -2.46875,
+ "stations": "Baily Enterprise,Harrington Settlement,Artin Relay,Riess Port,Wilhelm Landing"
+ },
+ {
+ "name": "Selkuntan",
+ "pos_x": 84.09375,
+ "pos_y": -239.09375,
+ "pos_z": 61.1875,
+ "stations": "Herschel Base"
+ },
+ {
+ "name": "Selkupath",
+ "pos_x": -123.34375,
+ "pos_y": -9.90625,
+ "pos_z": -31.46875,
+ "stations": "Potrykus Legacy"
+ },
+ {
+ "name": "Selniang",
+ "pos_x": 14.9375,
+ "pos_y": -8.03125,
+ "pos_z": -90.3125,
+ "stations": "Bakewell Terminal,Ramon Point"
+ },
+ {
+ "name": "Semakana",
+ "pos_x": -95,
+ "pos_y": -40.96875,
+ "pos_z": 20.3125,
+ "stations": "Wrangel Colony"
+ },
+ {
+ "name": "Semakhmets",
+ "pos_x": -104.84375,
+ "pos_y": -19.84375,
+ "pos_z": -61.09375,
+ "stations": "Garan Dock,Klimuk Market,Blodgett Enterprise,Joliot-Curie City,Horowitz Enterprise,Burnell Enterprise"
+ },
+ {
+ "name": "Semalanus",
+ "pos_x": 0.78125,
+ "pos_y": -128.5,
+ "pos_z": 107.28125,
+ "stations": "Phillips Port,Kirkwood Dock,Nilson Point,Peltier Vision,Medupe Observatory"
+ },
+ {
+ "name": "Semali",
+ "pos_x": 0.9375,
+ "pos_y": -56.9375,
+ "pos_z": 42.09375,
+ "stations": "Cartan Installation,Piserchia Dock,Rasch Hub"
+ },
+ {
+ "name": "Semayah",
+ "pos_x": 63.9375,
+ "pos_y": 1,
+ "pos_z": 84.25,
+ "stations": "Paulo da Gama Landing,Coney Camp,Sohl Orbital"
+ },
+ {
+ "name": "Sengen Sama",
+ "pos_x": 97.6875,
+ "pos_y": -1.71875,
+ "pos_z": 4.75,
+ "stations": "Barentsz Vision,Grimwood Orbital,Bujold Enterprise"
+ },
+ {
+ "name": "Sengu",
+ "pos_x": -4.8125,
+ "pos_y": -81.71875,
+ "pos_z": 87.75,
+ "stations": "Mikulin Platform,Maury Relay"
+ },
+ {
+ "name": "Senlu",
+ "pos_x": 9.90625,
+ "pos_y": -35.40625,
+ "pos_z": 25.875,
+ "stations": "Springer Gateway,Heng Dock,Kooi Port,Glashow Terminal,Townshend Enterprise,Farouk Silo"
+ },
+ {
+ "name": "Senocidi",
+ "pos_x": 78.8125,
+ "pos_y": -77.8125,
+ "pos_z": -139.59375,
+ "stations": "Hodgkin Dock,Szilard Enterprise,Dalmas Horizons,McCarthy Point"
+ },
+ {
+ "name": "Senones",
+ "pos_x": 5.15625,
+ "pos_y": -28.5625,
+ "pos_z": 66.84375,
+ "stations": "Jacquard Enterprise,Rennie Port,Akers Station,Edgeworth Enterprise,Dana Lab,Pascal Plant,Reisman Base,Ellison Prospect"
+ },
+ {
+ "name": "Senoni",
+ "pos_x": 51.46875,
+ "pos_y": 42.625,
+ "pos_z": -84.09375,
+ "stations": "Hope Enterprise,Lovell Port,Tarelkin Hub,Kummer Enterprise,Clapperton Vision,Whitson Market,Crown Terminal,Kuipers Port,Jun Orbital"
+ },
+ {
+ "name": "Sental",
+ "pos_x": 142.65625,
+ "pos_y": -217.59375,
+ "pos_z": 7.375,
+ "stations": "Seares Arena,Webb Vision"
+ },
+ {
+ "name": "Sentec",
+ "pos_x": -34.40625,
+ "pos_y": -120.78125,
+ "pos_z": 57.375,
+ "stations": "Yu Station,Gregory Station"
+ },
+ {
+ "name": "Sentei",
+ "pos_x": 77.90625,
+ "pos_y": -179.5,
+ "pos_z": -83.40625,
+ "stations": "Frechet Works,Harrison Holdings,Kidd Platform"
+ },
+ {
+ "name": "Senti",
+ "pos_x": 37.75,
+ "pos_y": 21.625,
+ "pos_z": 141.71875,
+ "stations": "al-Din Settlement,Read Depot"
+ },
+ {
+ "name": "Sentii",
+ "pos_x": 81.90625,
+ "pos_y": -21.59375,
+ "pos_z": 149.625,
+ "stations": "Pond Settlement,Tucker Orbital,Caselberg Orbital"
+ },
+ {
+ "name": "Sentinelese",
+ "pos_x": 15.71875,
+ "pos_y": 23.71875,
+ "pos_z": 135.5625,
+ "stations": "Haise Refinery,Aubakirov Point"
+ },
+ {
+ "name": "Senufan",
+ "pos_x": -2.40625,
+ "pos_y": -178.65625,
+ "pos_z": 13.28125,
+ "stations": "Dowie Arsenal,Reid Colony,Frazetta Terminal"
+ },
+ {
+ "name": "Senushan",
+ "pos_x": -37.40625,
+ "pos_y": 70.78125,
+ "pos_z": 131.46875,
+ "stations": "Bao Colony,Ponce de Leon Dock,Henderson Landing"
+ },
+ {
+ "name": "Senx",
+ "pos_x": 49.625,
+ "pos_y": 64.5,
+ "pos_z": 118.125,
+ "stations": "Lubin Port,Gordon Park,Chadwick Ring,Finch Depot,Pawelczyk Base"
+ },
+ {
+ "name": "Sepathians",
+ "pos_x": 145.65625,
+ "pos_y": 58.3125,
+ "pos_z": -61.65625,
+ "stations": "Clapperton Mine"
+ },
+ {
+ "name": "Septet",
+ "pos_x": 81.125,
+ "pos_y": -42.4375,
+ "pos_z": 37.21875,
+ "stations": "Kuo Refinery,Chacornac Beacon"
+ },
+ {
+ "name": "Sequichs",
+ "pos_x": -62.0625,
+ "pos_y": -48.125,
+ "pos_z": 109.3125,
+ "stations": "Karl Diesel Vision,Avdeyev Terminal,Godel Installation"
+ },
+ {
+ "name": "Serd",
+ "pos_x": 151,
+ "pos_y": -194,
+ "pos_z": -50.125,
+ "stations": "Zwicky Terminal,Kludze Colony,Vaucouleurs Vision,Stackpole Installation"
+ },
+ {
+ "name": "Serda",
+ "pos_x": 45.84375,
+ "pos_y": -4.40625,
+ "pos_z": 127.90625,
+ "stations": "White Colony,Hart Orbital,Manarov City"
+ },
+ {
+ "name": "Serishman",
+ "pos_x": 69.65625,
+ "pos_y": -183.21875,
+ "pos_z": 85.78125,
+ "stations": "Gehrels Vision"
+ },
+ {
+ "name": "Serklich",
+ "pos_x": -27.96875,
+ "pos_y": -84.03125,
+ "pos_z": 58.25,
+ "stations": "Mitchell Terminal,Adamson Station,Ross Hub,Soukup Base,Cori Dock,McCoy City,Szebehely Terminal,Kuttner's Inheritance,Gubarev Hub"
+ },
+ {
+ "name": "Serktomes",
+ "pos_x": 68.0625,
+ "pos_y": -55.15625,
+ "pos_z": -66.28125,
+ "stations": "Dann Orbital,Slonczewski Station,Al-Kashi City,Rand Enterprise,Powers Hub,Ford Dock,Sabine Terminal,Al Saud Palace,Forward Holdings,Godwin Station,Davidson Port,Bretnor Station,Macan Holdings"
+ },
+ {
+ "name": "Serquaesini",
+ "pos_x": 122.5,
+ "pos_y": -148.25,
+ "pos_z": -40.84375,
+ "stations": "Ashbrook Settlement"
+ },
+ {
+ "name": "Serque",
+ "pos_x": 15.40625,
+ "pos_y": 49.25,
+ "pos_z": -116.0625,
+ "stations": "Finney Vision,Delbruck Terminal,Clark Refinery"
+ },
+ {
+ "name": "Serqueret",
+ "pos_x": -119.53125,
+ "pos_y": -31.0625,
+ "pos_z": 95.46875,
+ "stations": "Herrington Hangar,Arber Relay"
+ },
+ {
+ "name": "Serra",
+ "pos_x": 93.9375,
+ "pos_y": -103.6875,
+ "pos_z": 112.75,
+ "stations": "Apianus Vision,Kingsbury Base"
+ },
+ {
+ "name": "Serre",
+ "pos_x": 79.53125,
+ "pos_y": 109.5,
+ "pos_z": 26.09375,
+ "stations": "Hermaszewski Orbital,Williamson Base,Williams Horizons"
+ },
+ {
+ "name": "Serret",
+ "pos_x": -44.5625,
+ "pos_y": -77.3125,
+ "pos_z": 66.78125,
+ "stations": "Kennan Horizons,Abasheli Ring"
+ },
+ {
+ "name": "Serrod",
+ "pos_x": 68.4375,
+ "pos_y": -200.875,
+ "pos_z": 18.53125,
+ "stations": "Payne's Folly,Eddington Station,Airy Settlement"
+ },
+ {
+ "name": "Serrot",
+ "pos_x": 19.15625,
+ "pos_y": -27.25,
+ "pos_z": 80.75,
+ "stations": "Addams Colony"
+ },
+ {
+ "name": "Seshenghus",
+ "pos_x": 68.15625,
+ "pos_y": -33.46875,
+ "pos_z": -22.125,
+ "stations": "Napier Camp,Rushd Platform"
+ },
+ {
+ "name": "Seshvaka",
+ "pos_x": 15.09375,
+ "pos_y": 96.03125,
+ "pos_z": 81.3125,
+ "stations": "Hunt Station,Beaufoy Dock,Nobel Enterprise,Oltion Hub"
+ },
+ {
+ "name": "Sesmeruku",
+ "pos_x": 164.5625,
+ "pos_y": 20,
+ "pos_z": 43.28125,
+ "stations": "Aldiss Port,Snodgrass Landing,Kozin Bastion"
+ },
+ {
+ "name": "Sesmetae",
+ "pos_x": 51.1875,
+ "pos_y": -25.25,
+ "pos_z": 55,
+ "stations": "Morgan Horizons"
+ },
+ {
+ "name": "Sesmu",
+ "pos_x": 180.84375,
+ "pos_y": -136.78125,
+ "pos_z": 6.1875,
+ "stations": "Galle Settlement"
+ },
+ {
+ "name": "Sesuang",
+ "pos_x": 45.6875,
+ "pos_y": -3.21875,
+ "pos_z": 22.125,
+ "stations": "Glazkov Depot,Meredith Vision,Drummond Enterprise,Stillman Terminal,Baturin Installation,Cook Legacy,Franklin Vision"
+ },
+ {
+ "name": "Sesui Redos",
+ "pos_x": 80.5,
+ "pos_y": -16.90625,
+ "pos_z": 99.3125,
+ "stations": "Reed Orbital,Laplace Penal colony,Savorgnan de Brazza City,Hiraga Enterprise,Brackett Prospect"
+ },
+ {
+ "name": "Sesur",
+ "pos_x": 138.09375,
+ "pos_y": -27.25,
+ "pos_z": 45.8125,
+ "stations": "Al Saud Gateway,Bella Port,Wollheim's Folly,Sargent's Claim,Berliner Dock"
+ },
+ {
+ "name": "Sesurakapu",
+ "pos_x": -3.53125,
+ "pos_y": -75.25,
+ "pos_z": 161.40625,
+ "stations": "Pullman Terminal,Coblentz Gateway,Sharp Keep"
+ },
+ {
+ "name": "Sesuvii",
+ "pos_x": -78.25,
+ "pos_y": -184.65625,
+ "pos_z": -5.21875,
+ "stations": "Brooks Settlement"
+ },
+ {
+ "name": "Sesuyar",
+ "pos_x": -42.875,
+ "pos_y": -31.65625,
+ "pos_z": 23.59375,
+ "stations": "al-Din Survey"
+ },
+ {
+ "name": "Setana",
+ "pos_x": -103.90625,
+ "pos_y": -39.40625,
+ "pos_z": -45.4375,
+ "stations": "Henson Horizons,Galiano Prospect,Ewald Installation"
+ },
+ {
+ "name": "Setati",
+ "pos_x": 151.90625,
+ "pos_y": 1.875,
+ "pos_z": 74.71875,
+ "stations": "Clerk Colony,Ross Works"
+ },
+ {
+ "name": "Sete",
+ "pos_x": 33.8125,
+ "pos_y": 43.34375,
+ "pos_z": -76.84375,
+ "stations": "Fox Dock,Bridges Horizons"
+ },
+ {
+ "name": "Setekh",
+ "pos_x": 71.75,
+ "pos_y": -224,
+ "pos_z": 46.65625,
+ "stations": "Swift Outpost"
+ },
+ {
+ "name": "Setesatsu",
+ "pos_x": 68.4375,
+ "pos_y": -112.65625,
+ "pos_z": 126.6875,
+ "stations": "Nomen Dock,Bessel Mines,Theodor Winnecke Vision,Napier Prospect"
+ },
+ {
+ "name": "Seteshaspi",
+ "pos_x": -119.71875,
+ "pos_y": -19.9375,
+ "pos_z": 87.65625,
+ "stations": "Williams Base,Shumil Reformatory"
+ },
+ {
+ "name": "Setesuyara",
+ "pos_x": -1.71875,
+ "pos_y": -101.09375,
+ "pos_z": 38.5625,
+ "stations": "Elcano Terminal,Chasles Vision,Dowling Station,Converse Orbital,Syromyatnikov Gateway"
+ },
+ {
+ "name": "Setet",
+ "pos_x": 18.96875,
+ "pos_y": -63.09375,
+ "pos_z": 155.125,
+ "stations": "Cerulli Port,Nagata Port,Knight Base,Butcher Settlement"
+ },
+ {
+ "name": "Setetynas",
+ "pos_x": -78.84375,
+ "pos_y": -91.8125,
+ "pos_z": 105.09375,
+ "stations": "Narbeth Stop,Green Survey"
+ },
+ {
+ "name": "Seurbi",
+ "pos_x": -62.1875,
+ "pos_y": -139.1875,
+ "pos_z": 0.59375,
+ "stations": "Trujillo Port,Lunan Enterprise,Bond Installation,Leydenfrost Ring"
+ },
+ {
+ "name": "Seurboda",
+ "pos_x": 83.03125,
+ "pos_y": -191.96875,
+ "pos_z": 120.46875,
+ "stations": "al-Kashi Camp"
+ },
+ {
+ "name": "Seurici",
+ "pos_x": 42.8125,
+ "pos_y": 30.25,
+ "pos_z": -147.5625,
+ "stations": "Buffett Depot,Helms Landing,Chilton Refinery"
+ },
+ {
+ "name": "Seurinohina",
+ "pos_x": -43.71875,
+ "pos_y": 108.09375,
+ "pos_z": -15.65625,
+ "stations": "Gurney Gateway,Bunch Enterprise,Ziljak Settlement"
+ },
+ {
+ "name": "Seurrion",
+ "pos_x": -26.0625,
+ "pos_y": -11.78125,
+ "pos_z": 98.28125,
+ "stations": "Fossey Terminal,Glazkov Gateway,Cooper City,Burkin Escape,Vaugh Enterprise,Marques Relay,Marusek Base"
+ },
+ {
+ "name": "Sevelitchit",
+ "pos_x": -126.4375,
+ "pos_y": 91.96875,
+ "pos_z": -56.40625,
+ "stations": "Ferguson Orbital,Herbert Orbital,McKee Vision,Norgay Lab,Cook Arsenal"
+ },
+ {
+ "name": "Severni",
+ "pos_x": -60.65625,
+ "pos_y": -102.96875,
+ "pos_z": -40.6875,
+ "stations": "Padalka Plant,Elion Installation"
+ },
+ {
+ "name": "Sha Di",
+ "pos_x": 58.3125,
+ "pos_y": 24.34375,
+ "pos_z": -78.375,
+ "stations": "Edgeworth Enterprise,Mayer Plant,Rukavishnikov Beacon,Dunn Hub"
+ },
+ {
+ "name": "Sha Gigas",
+ "pos_x": -35.875,
+ "pos_y": -66.5625,
+ "pos_z": 76.21875,
+ "stations": "Henize Orbital,Mayr Orbital"
+ },
+ {
+ "name": "Sha Wa",
+ "pos_x": 99.75,
+ "pos_y": -50.125,
+ "pos_z": -32.8125,
+ "stations": "Horowitz Dock,Scalzi Holdings,Haipeng Dock,Carson Hub"
+ },
+ {
+ "name": "Shabogama",
+ "pos_x": 122.125,
+ "pos_y": -24.9375,
+ "pos_z": 38.78125,
+ "stations": "Szameit Platform"
+ },
+ {
+ "name": "Shabos",
+ "pos_x": 137.625,
+ "pos_y": -103.03125,
+ "pos_z": 91.5625,
+ "stations": "Fowler Ring"
+ },
+ {
+ "name": "Shakal",
+ "pos_x": 114.15625,
+ "pos_y": 9,
+ "pos_z": 90.15625,
+ "stations": "Deere Port,Doi Station,Pausch Gateway,Noguchi Works"
+ },
+ {
+ "name": "Shakapa",
+ "pos_x": 52.5625,
+ "pos_y": -23.5,
+ "pos_z": 128.1875,
+ "stations": "Ore Horizons,Gibson Dock,Shapiro Escape,Chernykh Penal colony"
+ },
+ {
+ "name": "Shakar",
+ "pos_x": -87.375,
+ "pos_y": 4.9375,
+ "pos_z": -91.21875,
+ "stations": "Vian Prospect"
+ },
+ {
+ "name": "Shake",
+ "pos_x": 78.9375,
+ "pos_y": -16.25,
+ "pos_z": 97.8125,
+ "stations": "Neumann Plant,Bombelli Penal colony,Hill Colony"
+ },
+ {
+ "name": "Shakhe",
+ "pos_x": 87.0625,
+ "pos_y": 68.65625,
+ "pos_z": -85.09375,
+ "stations": "Wul's Progress"
+ },
+ {
+ "name": "Shakmbu",
+ "pos_x": 140.03125,
+ "pos_y": -101,
+ "pos_z": -63.40625,
+ "stations": "MacGill Horizons,Ilyushin Base,Muhammad Ibn Battuta Works"
+ },
+ {
+ "name": "Shakpana",
+ "pos_x": 22.96875,
+ "pos_y": 102.46875,
+ "pos_z": 90.0625,
+ "stations": "Abernathy Dock,Hawkes Platform,Malcolm Platform,Brunel Base,Hassanein Hub"
+ },
+ {
+ "name": "Shalatucas",
+ "pos_x": 85.09375,
+ "pos_y": 168.5625,
+ "pos_z": 46.375,
+ "stations": "Kiernan Co-operative,Diamond Port,Lowry Silo"
+ },
+ {
+ "name": "Shalit",
+ "pos_x": 88.34375,
+ "pos_y": 18.125,
+ "pos_z": 17.53125,
+ "stations": "Salk Ring,Wedge Gateway,Oxley Enterprise,Bosch Port,Wedge Hub,Selye Station,Casper Gateway,Leeuwenhoek Terminal,Teng Beacon,Dupuy de Lome Landing"
+ },
+ {
+ "name": "Shamaphan",
+ "pos_x": 179.21875,
+ "pos_y": -164.90625,
+ "pos_z": 71.875,
+ "stations": "Truman Station,Stromgren Platform,Biesbroeck Dock,Egan Installation"
+ },
+ {
+ "name": "Shamash",
+ "pos_x": 47.46875,
+ "pos_y": 28.28125,
+ "pos_z": -9.21875,
+ "stations": "Bierce Refinery,Garrett Orbital,Simak Keep"
+ },
+ {
+ "name": "Shamatana",
+ "pos_x": -42,
+ "pos_y": -15.0625,
+ "pos_z": 110.75,
+ "stations": "Irwin Port,Boulton Asylum,Dorsett Terminal"
+ },
+ {
+ "name": "Shambhala",
+ "pos_x": 48.5,
+ "pos_y": -134.8125,
+ "pos_z": -10.25,
+ "stations": "Neff Relay,Nomura Vision,Baynes City,Naddoddur's Inheritance,Harris Base"
+ },
+ {
+ "name": "Shambogi",
+ "pos_x": 75.9375,
+ "pos_y": -62.4375,
+ "pos_z": -8.90625,
+ "stations": "Adamson Works,Smith Works"
+ },
+ {
+ "name": "Shaminii",
+ "pos_x": 22.5625,
+ "pos_y": 62.8125,
+ "pos_z": -151.1875,
+ "stations": "Nicollet Terminal"
+ },
+ {
+ "name": "Shamo",
+ "pos_x": 127.4375,
+ "pos_y": -17.28125,
+ "pos_z": -49.53125,
+ "stations": "Wang Hub,Berliner Arsenal"
+ },
+ {
+ "name": "Shamor",
+ "pos_x": 53.90625,
+ "pos_y": 121.09375,
+ "pos_z": -55.6875,
+ "stations": "Arnold Port,Avdeyev Bastion"
+ },
+ {
+ "name": "Shampang",
+ "pos_x": -136.34375,
+ "pos_y": -71,
+ "pos_z": 45.1875,
+ "stations": "Thompson Landing,Andrews Settlement,Whitelaw Camp"
+ },
+ {
+ "name": "Shana Bei",
+ "pos_x": -72.1875,
+ "pos_y": -71.375,
+ "pos_z": -59.0625,
+ "stations": "Burbank Prospect,Barratt Hub,Chu City,Wetherbee Orbital,Ampere Station,Laue Station,Thomson Port,Mastracchio Port,Kirtley Installation,Bulychev Works"
+ },
+ {
+ "name": "Shanapi",
+ "pos_x": -47.3125,
+ "pos_y": -56.59375,
+ "pos_z": 122.875,
+ "stations": "Andersson Vision,Freycinet Reach"
+ },
+ {
+ "name": "Shang Wang",
+ "pos_x": -59.5,
+ "pos_y": 24.3125,
+ "pos_z": 113.78125,
+ "stations": "Watt Horizons,Jones Landing,Meyrink Hub"
+ },
+ {
+ "name": "Shangbe",
+ "pos_x": 193.875,
+ "pos_y": -126.3125,
+ "pos_z": 93.75,
+ "stations": "Pajdusakova Platform,Nomen Survey,Gottlob Frege Survey"
+ },
+ {
+ "name": "Shangdi",
+ "pos_x": -3.0625,
+ "pos_y": -50.84375,
+ "pos_z": -2.625,
+ "stations": "al-Din Platform,Becquerel Colony"
+ },
+ {
+ "name": "Shanhauls",
+ "pos_x": 83.53125,
+ "pos_y": -24.09375,
+ "pos_z": 94.5625,
+ "stations": "Lowry City,Forward Gateway"
+ },
+ {
+ "name": "Shankarns",
+ "pos_x": -39.5,
+ "pos_y": 95.1875,
+ "pos_z": 118.8125,
+ "stations": "Vogel Colony"
+ },
+ {
+ "name": "Shanluo Zi",
+ "pos_x": 29.1875,
+ "pos_y": -63.53125,
+ "pos_z": 92.09375,
+ "stations": "Fincke Hangar,Bose Landing"
+ },
+ {
+ "name": "Shanteneri",
+ "pos_x": 110.9375,
+ "pos_y": -113.0625,
+ "pos_z": 41.21875,
+ "stations": "Carnera Station,Norman Depot,Lilienthal Port"
+ },
+ {
+ "name": "Shany",
+ "pos_x": 60.40625,
+ "pos_y": 20.59375,
+ "pos_z": 147.34375,
+ "stations": "Houtman Vision,Pike Landing,Yolen Settlement,Fremion Market"
+ },
+ {
+ "name": "Shapa",
+ "pos_x": 2.34375,
+ "pos_y": -144.375,
+ "pos_z": 48.15625,
+ "stations": "Kushida Terminal,Stephan Terminal,Wilhelm von Struve Ring,Eisinga Landing"
+ },
+ {
+ "name": "Shapalam",
+ "pos_x": -128.4375,
+ "pos_y": 68.28125,
+ "pos_z": 100.125,
+ "stations": "Scalzi Dock,Baker Colony"
+ },
+ {
+ "name": "Shapanidoog",
+ "pos_x": -105.59375,
+ "pos_y": -77.46875,
+ "pos_z": -29.5,
+ "stations": "Ziemkiewicz Beacon,Maybury Refinery,Robson Installation"
+ },
+ {
+ "name": "Shapash",
+ "pos_x": 36.75,
+ "pos_y": 31.8125,
+ "pos_z": -19.375,
+ "stations": "Frick Refinery,Kraepelin Station,Jemison Settlement,Bulychev Installation"
+ },
+ {
+ "name": "Shaplini",
+ "pos_x": -112.5625,
+ "pos_y": -113.71875,
+ "pos_z": 2,
+ "stations": "Atwood Oasis,Bayley Colony"
+ },
+ {
+ "name": "Shaplinouk",
+ "pos_x": 47.375,
+ "pos_y": -244.6875,
+ "pos_z": 22,
+ "stations": "Arkhangelsky Enterprise,Beriev Platform,Fife Installation"
+ },
+ {
+ "name": "Shaplintin",
+ "pos_x": 137.0625,
+ "pos_y": -15,
+ "pos_z": -51.9375,
+ "stations": "Phillifent Vision,Ibold Landing,Ordway Horizons"
+ },
+ {
+ "name": "Shapori",
+ "pos_x": -130.5625,
+ "pos_y": -25.1875,
+ "pos_z": 92.78125,
+ "stations": "Kuo Camp"
+ },
+ {
+ "name": "Shapsugabus",
+ "pos_x": 91.78125,
+ "pos_y": -122.375,
+ "pos_z": 42.5,
+ "stations": "Biermann Station,Luther Terminal,Abe Dock,Balog Dock,Reinhold Dock,Lee Station,Nojiri City,Eddington Prospect,Melotte Prospect,Mikulin Installation,Kennicott Landing,Oosterhoff's Claim"
+ },
+ {
+ "name": "Shapsugs",
+ "pos_x": 155.28125,
+ "pos_y": -189.03125,
+ "pos_z": -36.84375,
+ "stations": "Shajn Settlement,Longomontanus Stop"
+ },
+ {
+ "name": "Sharakha",
+ "pos_x": -84.09375,
+ "pos_y": -21.125,
+ "pos_z": 63.53125,
+ "stations": "Carstensz Port,Bailey Vision,Narita Station,Greenland Keep"
+ },
+ {
+ "name": "Sharamo",
+ "pos_x": 30.3125,
+ "pos_y": -116.1875,
+ "pos_z": -72.71875,
+ "stations": "Slipher Terminal,Pinto Vista,al-Khowarizmi Depot"
+ },
+ {
+ "name": "Sharijio",
+ "pos_x": -39.375,
+ "pos_y": 35.96875,
+ "pos_z": -104.375,
+ "stations": "Vian Landing"
+ },
+ {
+ "name": "Sharru Sector FG-X b1-2",
+ "pos_x": 81.59375,
+ "pos_y": 75.40625,
+ "pos_z": -43.65625,
+ "stations": "Marques' Folly,Danvers Prospect"
+ },
+ {
+ "name": "Sharu",
+ "pos_x": -63.3125,
+ "pos_y": -33.59375,
+ "pos_z": 132.59375,
+ "stations": "Diophantus Terminal,de Caminha Mine"
+ },
+ {
+ "name": "Shasantei",
+ "pos_x": -19.28125,
+ "pos_y": 124.59375,
+ "pos_z": 99.78125,
+ "stations": "Agassiz Mines,Bass Enterprise"
+ },
+ {
+ "name": "Shasil",
+ "pos_x": 109.15625,
+ "pos_y": -82.84375,
+ "pos_z": -40.03125,
+ "stations": "Neujmin Station,Wollheim Platform,Kawasato Hangar,Taylor Mines"
+ },
+ {
+ "name": "Shasir",
+ "pos_x": -2.4375,
+ "pos_y": -92.65625,
+ "pos_z": -93.0625,
+ "stations": "Cummings Gateway,Conti Station,Steakley Hub,Shepard's Inheritance,Tolstoi's Folly,McArthur Base,Tarski Enterprise,Levy Ring"
+ },
+ {
+ "name": "Shastem",
+ "pos_x": 60.59375,
+ "pos_y": -204.4375,
+ "pos_z": 80.6875,
+ "stations": "Fan Terminal,Geller Enterprise,Daniel's Inheritance,Zamyatin Silo"
+ },
+ {
+ "name": "Shasti",
+ "pos_x": 61.0625,
+ "pos_y": 96.46875,
+ "pos_z": 66.625,
+ "stations": "Dukaj Colony,Eilenberg Prospect"
+ },
+ {
+ "name": "Shatist",
+ "pos_x": 92.03125,
+ "pos_y": 20.9375,
+ "pos_z": 123.125,
+ "stations": "Hodgkinson Beacon,Minkowski Survey"
+ },
+ {
+ "name": "Shatkwaka",
+ "pos_x": 27.4375,
+ "pos_y": -1.5,
+ "pos_z": 75.4375,
+ "stations": "Nasmyth Hub,Sevastyanov Station,Reightler Port"
+ },
+ {
+ "name": "Shatrites",
+ "pos_x": 92.34375,
+ "pos_y": -18.84375,
+ "pos_z": 52.125,
+ "stations": "Chomsky City,Coles Refinery,Hoard Horizons"
+ },
+ {
+ "name": "Shatrughna",
+ "pos_x": 126.21875,
+ "pos_y": -60.96875,
+ "pos_z": 89.9375,
+ "stations": "Bereznyak Orbital,Conway Relay"
+ },
+ {
+ "name": "Shattvasu",
+ "pos_x": 30.375,
+ "pos_y": -192.3125,
+ "pos_z": 79.90625,
+ "stations": "Jean Installation,Lundmark Depot"
+ },
+ {
+ "name": "Shebayeb",
+ "pos_x": -84.6875,
+ "pos_y": 55.71875,
+ "pos_z": -12.78125,
+ "stations": "Verrazzano's Progress,Kier Vision,Turtledove Mines"
+ },
+ {
+ "name": "Shebera",
+ "pos_x": 4.15625,
+ "pos_y": -39.3125,
+ "pos_z": -63.3125,
+ "stations": "Barratt Works"
+ },
+ {
+ "name": "Shebkauluwa",
+ "pos_x": 118.125,
+ "pos_y": -116.5,
+ "pos_z": 108.34375,
+ "stations": "Pilyugin Dock,Veron Port,Gustav Sporer Station,Lavochkin Station,Cyrrhus Terminal,Youll Terminal,Bradfield Orbital,Wells Vision"
+ },
+ {
+ "name": "Shedi",
+ "pos_x": -33.53125,
+ "pos_y": -19.90625,
+ "pos_z": -77.84375,
+ "stations": "Jahn Station,Sharma Hub,Korzun City,Great Palace,Forrester Station,Filipchenko City,Solovyev Ring,Hunt Legacy,Cormack Station,Wundt Exchange"
+ },
+ {
+ "name": "Sheela Na Gig",
+ "pos_x": -24.9375,
+ "pos_y": 67.46875,
+ "pos_z": -12.09375,
+ "stations": "Szilard Orbital,Krupkat Base,Mitchell Hub"
+ },
+ {
+ "name": "Shen Nung",
+ "pos_x": 50.21875,
+ "pos_y": -0.96875,
+ "pos_z": 112.4375,
+ "stations": "Lonchakov Relay,Khayyam Colony"
+ },
+ {
+ "name": "Shen Sali",
+ "pos_x": 58.3125,
+ "pos_y": -223.5625,
+ "pos_z": 15.15625,
+ "stations": "Dominique Depot,Arisman Stop"
+ },
+ {
+ "name": "Shen Tze",
+ "pos_x": 149.125,
+ "pos_y": 50.78125,
+ "pos_z": 42.09375,
+ "stations": "Teng-hui Outpost,Redi Landing"
+ },
+ {
+ "name": "Shen Yi",
+ "pos_x": 71.59375,
+ "pos_y": -14.90625,
+ "pos_z": 106.96875,
+ "stations": "Homer Terminal,Gloss Port,Wafer Hub"
+ },
+ {
+ "name": "Shencho",
+ "pos_x": 98.75,
+ "pos_y": -143.5,
+ "pos_z": 25.90625,
+ "stations": "Tombaugh Landing"
+ },
+ {
+ "name": "Shenet",
+ "pos_x": -15.28125,
+ "pos_y": -67.96875,
+ "pos_z": 100.78125,
+ "stations": "Sato Holdings,Polya Terminal,Anthony City,Vonarburg Orbital"
+ },
+ {
+ "name": "Shenetserii",
+ "pos_x": 15.21875,
+ "pos_y": -44.3125,
+ "pos_z": -33.4375,
+ "stations": "Aristotle Hub"
+ },
+ {
+ "name": "Sheng Hua",
+ "pos_x": 148.09375,
+ "pos_y": -174.71875,
+ "pos_z": -54,
+ "stations": "Lalande Colony,Dilworth Survey,Lewis Hub"
+ },
+ {
+ "name": "Sheng Norns",
+ "pos_x": 122.65625,
+ "pos_y": 99.125,
+ "pos_z": 103.5625,
+ "stations": "Vonarburg Holdings,Chaviano Horizons,Selberg Outpost,Almagro's Folly"
+ },
+ {
+ "name": "Shenggan",
+ "pos_x": 111.65625,
+ "pos_y": -11.34375,
+ "pos_z": 61.40625,
+ "stations": "Pasteur Dock,Grijalva Installation"
+ },
+ {
+ "name": "Shenich",
+ "pos_x": -26.09375,
+ "pos_y": -92.5625,
+ "pos_z": -31.28125,
+ "stations": "Wafer Terminal,Moresby Vision,De Lay Depot"
+ },
+ {
+ "name": "Shente",
+ "pos_x": 103.25,
+ "pos_y": -124.46875,
+ "pos_z": 60.0625,
+ "stations": "Eggen Terminal,Shklovsky Station,Staus Depot,Mead Ring,DiFate Gateway,Babcock Vision,Hawker Station,Bonestell Hub,Amedeo Plana Terminal,Foster City,Madsen Vista,Nilson Vision"
+ },
+ {
+ "name": "Shenteni",
+ "pos_x": -149.8125,
+ "pos_y": -55.65625,
+ "pos_z": 1.84375,
+ "stations": "Holland Vision,Lanier's Claim"
+ },
+ {
+ "name": "Shenufo",
+ "pos_x": 147.65625,
+ "pos_y": -113.375,
+ "pos_z": -5.96875,
+ "stations": "Johnson Arena,Hyakutake Depot"
+ },
+ {
+ "name": "Sherbo",
+ "pos_x": 20.21875,
+ "pos_y": -78.65625,
+ "pos_z": -74.9375,
+ "stations": "Albategnius Terminal,Baily Terminal,Bruck Port"
+ },
+ {
+ "name": "Shernaq",
+ "pos_x": -100.21875,
+ "pos_y": -0.46875,
+ "pos_z": 50.6875,
+ "stations": "Lenoir Prospect"
+ },
+ {
+ "name": "Shernteniai",
+ "pos_x": -72.25,
+ "pos_y": 117.34375,
+ "pos_z": -19.5,
+ "stations": "Phillips Station,Jiushao Hub,Lundwall Port,Hopkinson Station,Greenland Gateway,Polansky Barracks,Velho Port,Garden Survey,Clebsch Installation,Ordway Gateway,Clapperton Station,Tiedemann City"
+ },
+ {
+ "name": "Sherones",
+ "pos_x": 16.84375,
+ "pos_y": -128.8125,
+ "pos_z": 39,
+ "stations": "al-Din al-Urdi Orbital,Whitford Port,Sukhoi Terminal,Gustav Sporer Station,Salam Orbital,Goldreich Vision,Pajdusakova Terminal"
+ },
+ {
+ "name": "Sheroo",
+ "pos_x": 111.46875,
+ "pos_y": 37.4375,
+ "pos_z": 53.21875,
+ "stations": "Schirra Mines,Macquorn Rankine's Progress"
+ },
+ {
+ "name": "Shesmu",
+ "pos_x": 55.75,
+ "pos_y": 70.59375,
+ "pos_z": -57.6875,
+ "stations": "Ray Port,Baker Keep,Garay Enterprise"
+ },
+ {
+ "name": "Shi Yu",
+ "pos_x": 101.65625,
+ "pos_y": -92.875,
+ "pos_z": 59.78125,
+ "stations": "Hoffmeister Hub,Bode Port"
+ },
+ {
+ "name": "Shibboleth",
+ "pos_x": 8.125,
+ "pos_y": 17.125,
+ "pos_z": -59.46875,
+ "stations": "Elgin's Reach,Galiano Principality,Heaviside Landing"
+ },
+ {
+ "name": "Shili",
+ "pos_x": -84.53125,
+ "pos_y": 17.90625,
+ "pos_z": -75.78125,
+ "stations": "Mouhot Vision,al-Haytham's Claim,Stiegler Settlement"
+ },
+ {
+ "name": "Shilli",
+ "pos_x": -47.875,
+ "pos_y": -115.125,
+ "pos_z": 82.75,
+ "stations": "Hiyya Terminal,Delany Holdings,Nylund Plant,Pogson Orbital"
+ },
+ {
+ "name": "Shillici",
+ "pos_x": 190.125,
+ "pos_y": -177.4375,
+ "pos_z": -2.28125,
+ "stations": "Searle Relay"
+ },
+ {
+ "name": "Shilluk",
+ "pos_x": 84.28125,
+ "pos_y": -152.375,
+ "pos_z": -23,
+ "stations": "Stebbins Port,Pritchard Landing,Jansky Arsenal"
+ },
+ {
+ "name": "Shingon",
+ "pos_x": 121.6875,
+ "pos_y": -3.09375,
+ "pos_z": 47.1875,
+ "stations": "Hirn Port"
+ },
+ {
+ "name": "Shinigami",
+ "pos_x": 17.59375,
+ "pos_y": -122.96875,
+ "pos_z": 32.78125,
+ "stations": "Shone's Space Port,Andrew Yola"
+ },
+ {
+ "name": "Shinrarta Dezhra",
+ "pos_x": 55.71875,
+ "pos_y": 17.59375,
+ "pos_z": 27.15625,
+ "stations": "Jameson Memorial,Jameson Base,Gallun's Inheritance,Neumann Camp,Puleston Arsenal"
+ },
+ {
+ "name": "Shitici",
+ "pos_x": 18.21875,
+ "pos_y": 95.15625,
+ "pos_z": 16.84375,
+ "stations": "Bruce Hub,Neumann Enterprise,Weil Dock"
+ },
+ {
+ "name": "Shivarokkju",
+ "pos_x": 164.46875,
+ "pos_y": -4.84375,
+ "pos_z": 78.28125,
+ "stations": "Compton Relay,Narita Relay,Schouten Point"
+ },
+ {
+ "name": "Shivis",
+ "pos_x": -35.59375,
+ "pos_y": 40.53125,
+ "pos_z": -81.65625,
+ "stations": "Reiter Gateway,Usachov Hub,Bushkov Hub,Love Port"
+ },
+ {
+ "name": "Shojon",
+ "pos_x": 91.5625,
+ "pos_y": 94.71875,
+ "pos_z": 149.875,
+ "stations": "Stephens Stop,Palmer Base,Sturt Terminal"
+ },
+ {
+ "name": "Shoki Yine",
+ "pos_x": -26.75,
+ "pos_y": -130.09375,
+ "pos_z": -141.25,
+ "stations": "Morris Station,Reed Hub,Walker Settlement,Ziljak Beacon"
+ },
+ {
+ "name": "Shokondii",
+ "pos_x": 47.375,
+ "pos_y": -38.4375,
+ "pos_z": -59.5625,
+ "stations": "Matthews Dock,de Sousa Bastion,George Horizons"
+ },
+ {
+ "name": "Shoku",
+ "pos_x": 58.8125,
+ "pos_y": 111.46875,
+ "pos_z": 37.53125,
+ "stations": "Berezin Ring"
+ },
+ {
+ "name": "Shokudii",
+ "pos_x": -38.5625,
+ "pos_y": 70.25,
+ "pos_z": 134.28125,
+ "stations": "vo Platform"
+ },
+ {
+ "name": "Shokuju",
+ "pos_x": -59.75,
+ "pos_y": -158.34375,
+ "pos_z": 55.40625,
+ "stations": "Piano Orbital,Edwards Holdings,Arnold Schwassmann Dock,Elder Keep,Whymper Installation"
+ },
+ {
+ "name": "Shokuth",
+ "pos_x": -73.65625,
+ "pos_y": 128.625,
+ "pos_z": 5.125,
+ "stations": "Hyecho Port,Young Mines,Stillman Vision,Shalatula Gateway,Jordan Settlement"
+ },
+ {
+ "name": "Shokwa",
+ "pos_x": 15.15625,
+ "pos_y": -33.125,
+ "pos_z": -41.0625,
+ "stations": "Burnet Hub"
+ },
+ {
+ "name": "Shokwakw",
+ "pos_x": 20.78125,
+ "pos_y": 1.46875,
+ "pos_z": 119.8125,
+ "stations": "Maudslay Platform,Parmitano Plant"
+ },
+ {
+ "name": "Shokwus",
+ "pos_x": -6.28125,
+ "pos_y": -148.5625,
+ "pos_z": -5.6875,
+ "stations": "White Prospect,Whipple Survey,Jones Survey"
+ },
+ {
+ "name": "Shol",
+ "pos_x": 52.65625,
+ "pos_y": -162.34375,
+ "pos_z": -84.8125,
+ "stations": "Wilhelm Survey,Aitken Outpost,Lagrange Bastion"
+ },
+ {
+ "name": "Sholintet",
+ "pos_x": -150.09375,
+ "pos_y": -73.40625,
+ "pos_z": -16.6875,
+ "stations": "Brandenstein Works,Galilei Asylum"
+ },
+ {
+ "name": "Shom Djero",
+ "pos_x": 107.625,
+ "pos_y": -140.59375,
+ "pos_z": 26.40625,
+ "stations": "Alphonsi Colony,Eisinga Vision"
+ },
+ {
+ "name": "Shom Du",
+ "pos_x": 163.9375,
+ "pos_y": -148.53125,
+ "pos_z": 74.75,
+ "stations": "Marriott Relay"
+ },
+ {
+ "name": "Shom Dun",
+ "pos_x": 53.5,
+ "pos_y": 44.3125,
+ "pos_z": -77.59375,
+ "stations": "Tudela Point,Sarafanov Station,Xiaoguan Hub"
+ },
+ {
+ "name": "Shoma",
+ "pos_x": 71.8125,
+ "pos_y": -218.28125,
+ "pos_z": -55.78125,
+ "stations": "Perlmutter Arena,Hooker Mine"
+ },
+ {
+ "name": "Shomanjia",
+ "pos_x": 94.34375,
+ "pos_y": -122.46875,
+ "pos_z": -63.6875,
+ "stations": "Hayashi Landing,Jansky Colony,Penrose Plant,Alexander Holdings"
+ },
+ {
+ "name": "Shon",
+ "pos_x": -48.15625,
+ "pos_y": -120.9375,
+ "pos_z": -7.625,
+ "stations": "Kennan Colony,Dunyach Colony,Cook Depot,Aikin Survey,Chertovsky Prospect"
+ },
+ {
+ "name": "Shona",
+ "pos_x": -160.625,
+ "pos_y": 5.84375,
+ "pos_z": -62.96875,
+ "stations": "Abbott Landing,Moran City,Cramer Enterprise,Wnuk-Lipinski Landing,Crichton Observatory"
+ },
+ {
+ "name": "Shonessa",
+ "pos_x": -40.09375,
+ "pos_y": -174.625,
+ "pos_z": 17.09375,
+ "stations": "Herzog City,Roelofs City,Jones Vision,Gaiman Beacon"
+ },
+ {
+ "name": "Shongastyak",
+ "pos_x": 84.15625,
+ "pos_y": -101.9375,
+ "pos_z": -27.25,
+ "stations": "Gwynn Penitentiary,Wait Colburn's Claim"
+ },
+ {
+ "name": "Shongbon",
+ "pos_x": 87.15625,
+ "pos_y": -50.9375,
+ "pos_z": 7.0625,
+ "stations": "Bernoulli Landing,de Kamp Enterprise,Fanning Port"
+ },
+ {
+ "name": "Shono",
+ "pos_x": 57.4375,
+ "pos_y": -16.625,
+ "pos_z": -121.3125,
+ "stations": "Russ Dock,Rodrigues Colony,Tiedemann Settlement"
+ },
+ {
+ "name": "Shonpono",
+ "pos_x": -78.5625,
+ "pos_y": -82.3125,
+ "pos_z": -3.40625,
+ "stations": "Pelt Prospect,Tucker Enterprise,Nourse Ring,Russo Bastion"
+ },
+ {
+ "name": "Shonso",
+ "pos_x": 64.3125,
+ "pos_y": -137.15625,
+ "pos_z": -29.40625,
+ "stations": "Hill Tinsley Station,Rechtin Ring,Witt Gateway,Napier Lab,Schilling Survey"
+ },
+ {
+ "name": "Shontii",
+ "pos_x": -39.3125,
+ "pos_y": -119.25,
+ "pos_z": -23.5625,
+ "stations": "Bohrmann Vision,Webb Terminal,Bridger Terminal,Darnielle Barracks"
+ },
+ {
+ "name": "Shonto",
+ "pos_x": 47.34375,
+ "pos_y": 68.3125,
+ "pos_z": -53.90625,
+ "stations": "Lonchakov Port,Snyder Colony,Boming Mines"
+ },
+ {
+ "name": "Shoribelu",
+ "pos_x": -3.4375,
+ "pos_y": 23.78125,
+ "pos_z": -161.71875,
+ "stations": "Johnson Dock,Coulomb Prospect,Jordan Station,Ivens Enterprise"
+ },
+ {
+ "name": "Shorinans",
+ "pos_x": -41.1875,
+ "pos_y": 32.65625,
+ "pos_z": 166.09375,
+ "stations": "Poncelet Platform,Barjavel Depot"
+ },
+ {
+ "name": "Shorix",
+ "pos_x": 80.96875,
+ "pos_y": -41.03125,
+ "pos_z": -63,
+ "stations": "Garriott Installation"
+ },
+ {
+ "name": "Shorodo",
+ "pos_x": 159.40625,
+ "pos_y": -253.5625,
+ "pos_z": 99.3125,
+ "stations": "Akiyama Hub,Baillaud Orbital,Kimura Orbital"
+ },
+ {
+ "name": "Shorong",
+ "pos_x": -136.15625,
+ "pos_y": -11.28125,
+ "pos_z": 86.4375,
+ "stations": "Murray Vision"
+ },
+ {
+ "name": "Shoroteni",
+ "pos_x": -87.46875,
+ "pos_y": -13.1875,
+ "pos_z": -133.90625,
+ "stations": "Crown Dock,Hartlib Park,Wescott Settlement,de Caminha Beacon"
+ },
+ {
+ "name": "Shos",
+ "pos_x": 125.4375,
+ "pos_y": -21.09375,
+ "pos_z": -42.3125,
+ "stations": "Williams Enterprise,Al-Farabi Colony,Fairbairn Hangar"
+ },
+ {
+ "name": "Shosak",
+ "pos_x": -40.6875,
+ "pos_y": 35.84375,
+ "pos_z": 140.46875,
+ "stations": "Afanasyev Orbital,Jernigan City,Tarelkin Platform,Luiken Base"
+ },
+ {
+ "name": "Shosha",
+ "pos_x": -5.34375,
+ "pos_y": -43.25,
+ "pos_z": 81.53125,
+ "stations": "Wigura Orbital,de Sousa Legacy"
+ },
+ {
+ "name": "Shoshonean",
+ "pos_x": 57.1875,
+ "pos_y": 36.96875,
+ "pos_z": -144.65625,
+ "stations": "Judson Survey,Baffin Point,Offutt Base"
+ },
+ {
+ "name": "Shosini",
+ "pos_x": 168.84375,
+ "pos_y": -111.25,
+ "pos_z": 38.3125,
+ "stations": "Valigursky Bastion,Kludze Enterprise"
+ },
+ {
+ "name": "Shotel",
+ "pos_x": 34.15625,
+ "pos_y": -201.78125,
+ "pos_z": 128.625,
+ "stations": "Bharadwaj Stop,Nomen Colony,Cousteau's Progress"
+ },
+ {
+ "name": "Shotep",
+ "pos_x": -58.125,
+ "pos_y": 9.84375,
+ "pos_z": 90.5625,
+ "stations": "Butcher Orbital,Walters Refinery,Fu Installation"
+ },
+ {
+ "name": "Shottunche",
+ "pos_x": -59.84375,
+ "pos_y": 55.875,
+ "pos_z": -82.40625,
+ "stations": "Jones Dock,Brendan Depot"
+ },
+ {
+ "name": "Shou",
+ "pos_x": 135.71875,
+ "pos_y": 43.59375,
+ "pos_z": 63.9375,
+ "stations": "Steele Mine,Ehrenfried Kegel Holdings"
+ },
+ {
+ "name": "Shou Mao",
+ "pos_x": -13.5625,
+ "pos_y": -118.84375,
+ "pos_z": 100.84375,
+ "stations": "Antonov Installation,Lozino-Lozinskiy Holdings,Smirnova's Folly"
+ },
+ {
+ "name": "Shou Wu Gu",
+ "pos_x": 66.5,
+ "pos_y": -121.40625,
+ "pos_z": 101.09375,
+ "stations": "Artsutanov Estate,Kahn Refinery,Reinhold's Folly,Schumacher Plant"
+ },
+ {
+ "name": "Shou Wuru",
+ "pos_x": -68.8125,
+ "pos_y": 53.09375,
+ "pos_z": 118.46875,
+ "stations": "Wood Installation,Fuca Plant"
+ },
+ {
+ "name": "Shou Xing",
+ "pos_x": -16.28125,
+ "pos_y": -44.53125,
+ "pos_z": 94.375,
+ "stations": "Shargin Gateway"
+ },
+ {
+ "name": "Shoujeman",
+ "pos_x": 12.4375,
+ "pos_y": 30.90625,
+ "pos_z": 9.15625,
+ "stations": "Binnie Orbital,Hertz Refinery,Zalyotin Dock,Baffin Plant,Scheerbart's Folly"
+ },
+ {
+ "name": "Shouvul",
+ "pos_x": 28.53125,
+ "pos_y": 17.875,
+ "pos_z": -88.90625,
+ "stations": "Dutton Enterprise,Brady City,Nicollier Port,Locke Terminal,Aikin Silo"
+ },
+ {
+ "name": "Shu Babassi",
+ "pos_x": -2.5625,
+ "pos_y": -264.46875,
+ "pos_z": 22.65625,
+ "stations": "Matthews Mines,Schoening Base"
+ },
+ {
+ "name": "Shu Jinga",
+ "pos_x": 53.78125,
+ "pos_y": -34.96875,
+ "pos_z": 114.375,
+ "stations": "Boltzmann Base"
+ },
+ {
+ "name": "Shu Rong",
+ "pos_x": 53.5,
+ "pos_y": 19.53125,
+ "pos_z": -113.25,
+ "stations": "Manarov City,Thuot Port,Meucci Port,Gaffney Orbital,Surayev Port,Onufriyenko Terminal,Laue Dock,Laird's Claim,Wisniewski-Snerg Port"
+ },
+ {
+ "name": "Shui",
+ "pos_x": 88.1875,
+ "pos_y": -237.6875,
+ "pos_z": -8.0625,
+ "stations": "Sudworth Survey,Isaev's Pride,Nagata Silo"
+ },
+ {
+ "name": "Shuilela",
+ "pos_x": -62.875,
+ "pos_y": -101.5625,
+ "pos_z": 116.5,
+ "stations": "Midgeley Enterprise,Ricardo Gateway,Hughes-Fulford Gateway,Nicholson Colony"
+ },
+ {
+ "name": "Shuitabraki",
+ "pos_x": 161.5625,
+ "pos_y": -125.53125,
+ "pos_z": -69.5625,
+ "stations": "Naubakht Platform,Wolszczan Survey,Hagihara's Progress"
+ },
+ {
+ "name": "Shun Ceht",
+ "pos_x": -142.09375,
+ "pos_y": -33.125,
+ "pos_z": 75.625,
+ "stations": "Starzl Installation"
+ },
+ {
+ "name": "Shuna",
+ "pos_x": 51.78125,
+ "pos_y": -94.625,
+ "pos_z": -53.21875,
+ "stations": "Hedin Survey,Ponce de Leon Arena"
+ },
+ {
+ "name": "Si Langul",
+ "pos_x": 31.1875,
+ "pos_y": -181.4375,
+ "pos_z": 32.1875,
+ "stations": "Whittle Dock,Yano Horizons,Pickering City,Cantor Arsenal"
+ },
+ {
+ "name": "Si Lao",
+ "pos_x": 76.0625,
+ "pos_y": -42.96875,
+ "pos_z": 134.9375,
+ "stations": "Hill Terminal"
+ },
+ {
+ "name": "Si Lisu",
+ "pos_x": -47.5,
+ "pos_y": -85.125,
+ "pos_z": 72.25,
+ "stations": "Chu Settlement,Nelder Horizons,Thorne Settlement"
+ },
+ {
+ "name": "Siaroju",
+ "pos_x": 86.28125,
+ "pos_y": -20.9375,
+ "pos_z": 100.21875,
+ "stations": "Cabral Asylum,Wright Works"
+ },
+ {
+ "name": "Siaronia",
+ "pos_x": 155.71875,
+ "pos_y": -111.78125,
+ "pos_z": -58.5,
+ "stations": "Langley Camp,Barreiros Base,Kidd Depot"
+ },
+ {
+ "name": "Sibi",
+ "pos_x": -45.21875,
+ "pos_y": -42.71875,
+ "pos_z": 101.5,
+ "stations": "Anderson Platform,Kandel Horizons,Parry Settlement"
+ },
+ {
+ "name": "Sibia",
+ "pos_x": -138.15625,
+ "pos_y": 37.21875,
+ "pos_z": -100.84375,
+ "stations": "Yang Platform,Loncke Vision,Oefelein Port,Keyes Settlement"
+ },
+ {
+ "name": "Sibianoe",
+ "pos_x": 35.59375,
+ "pos_y": -183.4375,
+ "pos_z": -55.0625,
+ "stations": "Impey Ring,Barnes' Claim,Bauschinger Gateway"
+ },
+ {
+ "name": "Sibil",
+ "pos_x": 74.40625,
+ "pos_y": -131.84375,
+ "pos_z": -1.28125,
+ "stations": "Salpeter Vision,Very Station"
+ },
+ {
+ "name": "Sibilja",
+ "pos_x": 106.65625,
+ "pos_y": -90.65625,
+ "pos_z": -39.875,
+ "stations": "Freas Dock,Hay Orbital,Park Installation,Palitzsch Port,Beaufoy Prospect"
+ },
+ {
+ "name": "Sibiljada",
+ "pos_x": 15.09375,
+ "pos_y": 89.375,
+ "pos_z": 137.03125,
+ "stations": "Nicollet Colony"
+ },
+ {
+ "name": "Sibio",
+ "pos_x": 11.09375,
+ "pos_y": -89.21875,
+ "pos_z": 121,
+ "stations": "d'Arrest Orbital"
+ },
+ {
+ "name": "Sibisius",
+ "pos_x": -43.78125,
+ "pos_y": 97.65625,
+ "pos_z": -56.1875,
+ "stations": "Bethke Port"
+ },
+ {
+ "name": "Sibitia",
+ "pos_x": -37.0625,
+ "pos_y": 81.625,
+ "pos_z": 103.9375,
+ "stations": "Zholobov Platform"
+ },
+ {
+ "name": "Sibitica",
+ "pos_x": 85.90625,
+ "pos_y": 142.28125,
+ "pos_z": 51.78125,
+ "stations": "Webb Orbital,Bass City,Harrison Colony,Chaviano Lab,Robinson Holdings"
+ },
+ {
+ "name": "Sibito",
+ "pos_x": 48.6875,
+ "pos_y": -79.8125,
+ "pos_z": -124.21875,
+ "stations": "Creamer Orbital,Ferguson Dock,Serebrov Enterprise,Hertz Orbital"
+ },
+ {
+ "name": "Siculottii",
+ "pos_x": 169.96875,
+ "pos_y": -131.6875,
+ "pos_z": 8.46875,
+ "stations": "Oren's Progress,Suzuki Survey"
+ },
+ {
+ "name": "Sicuncheim",
+ "pos_x": 93.78125,
+ "pos_y": -116,
+ "pos_z": 43.375,
+ "stations": "Draper Market,Mille Installation"
+ },
+ {
+ "name": "Sicusha",
+ "pos_x": 4.46875,
+ "pos_y": 44.65625,
+ "pos_z": 169.28125,
+ "stations": "McQuay Installation,Bulmer Point"
+ },
+ {
+ "name": "Siddha",
+ "pos_x": 24.59375,
+ "pos_y": -108.96875,
+ "pos_z": -4.0625,
+ "stations": "Blaauw Orbital,Meuron Station,Gaensler Prospect,Siddha Port,Ludwig Struve Hub,Wallerstein Terminal,Aaronson City,Langley Dock,Wyndham Landing,Gunter Station,Otomo Market"
+ },
+ {
+ "name": "Siddhas",
+ "pos_x": 92.28125,
+ "pos_y": -55.9375,
+ "pos_z": 99.375,
+ "stations": "Bauman Mines,Jeans Holdings,Hickman's Claim"
+ },
+ {
+ "name": "Siddi Di",
+ "pos_x": 99.78125,
+ "pos_y": -103.84375,
+ "pos_z": -101.1875,
+ "stations": "Farrukh Keep,Mustelin Mine"
+ },
+ {
+ "name": "Siddion",
+ "pos_x": 79.46875,
+ "pos_y": -32.59375,
+ "pos_z": 111.28125,
+ "stations": "Pike Lab,Piccard Relay,Great Terminal"
+ },
+ {
+ "name": "Sieganga",
+ "pos_x": 36.25,
+ "pos_y": -147.21875,
+ "pos_z": -22.46875,
+ "stations": "Gunter Dock,Griffiths Survey,Pilcher Vision,Bolkow Landing,Ziegler Terminal"
+ },
+ {
+ "name": "Siegfries",
+ "pos_x": -129.90625,
+ "pos_y": -22.09375,
+ "pos_z": -24.375,
+ "stations": "Rucker Point,Keyes Point,Nachtigal Legacy"
+ },
+ {
+ "name": "Sieni",
+ "pos_x": 22.8125,
+ "pos_y": -24.78125,
+ "pos_z": -160.375,
+ "stations": "Malcolm Point"
+ },
+ {
+ "name": "Sienses",
+ "pos_x": -82.21875,
+ "pos_y": -17.375,
+ "pos_z": -60.8125,
+ "stations": "Hackworth Mine"
+ },
+ {
+ "name": "Sietae",
+ "pos_x": 27.6875,
+ "pos_y": -80.5,
+ "pos_z": -101,
+ "stations": "Nakamura Orbital,Haack Orbital,Smirnova Orbital,Nahavandi City,Shklovsky Vision,Mayer Arsenal,Mitra Terminal,McMahon Installation,Laird Point,Al-Kashi Holdings,Manning Vision,Ulloa Hub,Lassell Dock"
+ },
+ {
+ "name": "Sietynas",
+ "pos_x": 72.6875,
+ "pos_y": 27.65625,
+ "pos_z": 137.6875,
+ "stations": "Redi Base,Titov Sanctuary"
+ },
+ {
+ "name": "Sif",
+ "pos_x": 103.25,
+ "pos_y": -141.46875,
+ "pos_z": 65.28125,
+ "stations": "Denning Station,Michalitsianos Silo,Biruni Ring,Godwin Installation,Darwin Colony"
+ },
+ {
+ "name": "Sifjar",
+ "pos_x": 10.15625,
+ "pos_y": -82.84375,
+ "pos_z": 42.21875,
+ "stations": "Isherwood Settlement,Graham Hangar,Herschel Landing,Abe Works,Hedin Survey,Mobius Platform"
+ },
+ {
+ "name": "Sigma Andromedae",
+ "pos_x": -109.25,
+ "pos_y": -57.84375,
+ "pos_z": -53.78125,
+ "stations": "Carleson City,Blaylock Relay"
+ },
+ {
+ "name": "Sigma Bootis",
+ "pos_x": -14.46875,
+ "pos_y": 47.4375,
+ "pos_z": 14.40625,
+ "stations": "Chaudhary City,Collins Gateway,Vetulani City,Onufriyenko Hub,Palmer Survey,Marusek Enterprise,Beaumont Base"
+ },
+ {
+ "name": "Sigma Geminorum",
+ "pos_x": 22.90625,
+ "pos_y": 50.59375,
+ "pos_z": -112.0625,
+ "stations": "Fulton City"
+ },
+ {
+ "name": "Sigma Hydri",
+ "pos_x": 90.90625,
+ "pos_y": -82.46875,
+ "pos_z": 49.84375,
+ "stations": "DamnClown's Funland,Asire Orbital,Bunch Terminal,Mike Tapa Astronautics Ltd"
+ },
+ {
+ "name": "Sigma Pegasi",
+ "pos_x": -64.125,
+ "pos_y": -61,
+ "pos_z": 9.25,
+ "stations": "Zetford Orbital,Hume Orbital,Cadamosto Market,Swanwick Hub,Hogan Enterprise,Betancourt Ring,Blackman City,Harris Port,VanderMeer Enterprise,Rothman's Progress,Herodotus Legacy,Keyes Beacon"
+ },
+ {
+ "name": "Sigma Puppis",
+ "pos_x": 174.65625,
+ "pos_y": -37.3125,
+ "pos_z": -43.6875,
+ "stations": "Mitchell Beacon"
+ },
+ {
+ "name": "Sigma Sculptoris",
+ "pos_x": 20.53125,
+ "pos_y": -231.40625,
+ "pos_z": -0.5,
+ "stations": "Cooper Installation,Sitterly Hub,Faye Dock"
+ },
+ {
+ "name": "Sigma Serpentis",
+ "pos_x": -19.5,
+ "pos_y": 47.9375,
+ "pos_z": 72.3125,
+ "stations": "Sinclair's Claim"
+ },
+ {
+ "name": "Sigma-2 Gruis",
+ "pos_x": 2.875,
+ "pos_y": -185.71875,
+ "pos_z": 107.75,
+ "stations": "Florine Settlement,Babakin Hub"
+ },
+ {
+ "name": "Sigma-2 Ursae Majoris",
+ "pos_x": -28.375,
+ "pos_y": 41.4375,
+ "pos_z": -43.5625,
+ "stations": "Artin Dock,Fermat Gateway,Brust Ring"
+ },
+ {
+ "name": "Signalis",
+ "pos_x": -9509.75,
+ "pos_y": -916.8125,
+ "pos_z": 19788.53125,
+ "stations": "Broadcasting Bay"
+ },
+ {
+ "name": "Sigru",
+ "pos_x": 37.6875,
+ "pos_y": -9.90625,
+ "pos_z": -102.65625,
+ "stations": "Hernandez Gateway,Clair Prospect,Bulgakov Palace,Hartsfield Landing,H. G. Wells Base,Faraday Terminal"
+ },
+ {
+ "name": "Sigue",
+ "pos_x": 128.40625,
+ "pos_y": -222.15625,
+ "pos_z": -58.40625,
+ "stations": "Ptack Dock,Bering Plant,Smyth Landing,Rasmussen Plant"
+ },
+ {
+ "name": "Sigur",
+ "pos_x": -74.8125,
+ "pos_y": -120.15625,
+ "pos_z": -81.75,
+ "stations": "Vetulani Terminal"
+ },
+ {
+ "name": "Sigurd",
+ "pos_x": 57.0625,
+ "pos_y": -122,
+ "pos_z": 56.4375,
+ "stations": "Chaffee Camp"
+ },
+ {
+ "name": "Sigynnae",
+ "pos_x": 135.1875,
+ "pos_y": -29.8125,
+ "pos_z": 88.9375,
+ "stations": "Effinger Mines"
+ },
+ {
+ "name": "Sigynnarsu",
+ "pos_x": -128.5625,
+ "pos_y": 19.4375,
+ "pos_z": 114.3125,
+ "stations": "Henricks Platform"
+ },
+ {
+ "name": "Sikarici",
+ "pos_x": 29.21875,
+ "pos_y": 82.09375,
+ "pos_z": 8,
+ "stations": "Haldeman II Settlement,Yegorov's Folly,Manning Port,Favier Penal colony"
+ },
+ {
+ "name": "Siki",
+ "pos_x": 84.71875,
+ "pos_y": 62.34375,
+ "pos_z": 27.25,
+ "stations": "Kent's Inheritance,Lee Vision"
+ },
+ {
+ "name": "Sikiani",
+ "pos_x": 136.96875,
+ "pos_y": -33.03125,
+ "pos_z": -50.78125,
+ "stations": "Kurtz Horizons,Dhawan Hub"
+ },
+ {
+ "name": "Sikians",
+ "pos_x": 35.65625,
+ "pos_y": -29.4375,
+ "pos_z": 0.75,
+ "stations": "Rondon Landing,Zetford Hub"
+ },
+ {
+ "name": "Sikitoq",
+ "pos_x": 0.8125,
+ "pos_y": 99.4375,
+ "pos_z": -143.84375,
+ "stations": "Oluwafemi Horizons,Yang Vision"
+ },
+ {
+ "name": "Sikkai",
+ "pos_x": -51.875,
+ "pos_y": -169.1875,
+ "pos_z": 59.3125,
+ "stations": "Sugano Hub,Stechkin Gateway,Chadwick Orbital,Heck Forum,Bischoff Bastion"
+ },
+ {
+ "name": "Sikshmi",
+ "pos_x": -41.78125,
+ "pos_y": -155.75,
+ "pos_z": 57.5625,
+ "stations": "Kuiper's Claim"
+ },
+ {
+ "name": "Siksikabe",
+ "pos_x": 115.625,
+ "pos_y": 86.96875,
+ "pos_z": 85.03125,
+ "stations": "Potrykus Gateway,Linge Prospect,Yeliseyev Arsenal"
+ },
+ {
+ "name": "Siksikas",
+ "pos_x": 105.09375,
+ "pos_y": -140.78125,
+ "pos_z": -13.96875,
+ "stations": "Baily Terminal,Wilson Dock,Derekas Enterprise,Smith Survey,Nomura Settlement"
+ },
+ {
+ "name": "Silinja",
+ "pos_x": 99.1875,
+ "pos_y": -4.71875,
+ "pos_z": 109.125,
+ "stations": "Onufriyenko Hub,Messerschmid Landing,Needham Horizons"
+ },
+ {
+ "name": "Silinqang",
+ "pos_x": 124.53125,
+ "pos_y": -174.34375,
+ "pos_z": 75,
+ "stations": "Addams Mines,Gutierrez Vision"
+ },
+ {
+ "name": "Silintae",
+ "pos_x": -145.4375,
+ "pos_y": -84.53125,
+ "pos_z": 106.65625,
+ "stations": "Lorrah Dock,Janes Settlement"
+ },
+ {
+ "name": "Siliwuarisu",
+ "pos_x": 106.0625,
+ "pos_y": -205.6875,
+ "pos_z": -13.03125,
+ "stations": "Mooz Platform,Gould Survey,Friedrich Peters Silo,Maddox Sanctuary"
+ },
+ {
+ "name": "Silurinmuti",
+ "pos_x": 103.75,
+ "pos_y": -199.46875,
+ "pos_z": -37.34375,
+ "stations": "Vyssotsky Stop"
+ },
+ {
+ "name": "Silurion",
+ "pos_x": 76.75,
+ "pos_y": 53.15625,
+ "pos_z": 153.9375,
+ "stations": "Carstensz Port,King Observatory"
+ },
+ {
+ "name": "Siluror",
+ "pos_x": -28.65625,
+ "pos_y": -97.75,
+ "pos_z": 63.6875,
+ "stations": "Reed Station,Abel Terminal,Baxter Port,Popov Hub,Dupuy de Lome's Pride,Knight Hub,Darlton's Claim,Hiroyuki City,Burstein Terminal,Reinhold Settlement,Witt Observatory"
+ },
+ {
+ "name": "Siluruba",
+ "pos_x": -0.125,
+ "pos_y": 140.46875,
+ "pos_z": -22.90625,
+ "stations": "Stevens Dock"
+ },
+ {
+ "name": "Silvait",
+ "pos_x": 155.09375,
+ "pos_y": -116.4375,
+ "pos_z": 25.1875,
+ "stations": "Penzias Terminal"
+ },
+ {
+ "name": "Silvandumal",
+ "pos_x": -121.3125,
+ "pos_y": 16.65625,
+ "pos_z": -79.625,
+ "stations": "Stephenson Landing,Hirase Horizons"
+ },
+ {
+ "name": "Silvandza",
+ "pos_x": -101.21875,
+ "pos_y": -49.71875,
+ "pos_z": -50.5625,
+ "stations": "Shonin Enterprise,Ramanujan Station"
+ },
+ {
+ "name": "Silvane",
+ "pos_x": 79.90625,
+ "pos_y": 52.6875,
+ "pos_z": -98.25,
+ "stations": "Cartmill Vision"
+ },
+ {
+ "name": "Silvanitou",
+ "pos_x": 5.625,
+ "pos_y": -29.53125,
+ "pos_z": 11.5625,
+ "stations": "Evans Terminal,Buffett Platform,Solovyev Station"
+ },
+ {
+ "name": "Silvantzin",
+ "pos_x": -3.15625,
+ "pos_y": -8.25,
+ "pos_z": 96.75,
+ "stations": "Hewish Colony,Leclerc Base"
+ },
+ {
+ "name": "Simarasir",
+ "pos_x": -36.25,
+ "pos_y": -73.65625,
+ "pos_z": -89.3125,
+ "stations": "Hawking Point,Cabot Observatory,Dutton Base"
+ },
+ {
+ "name": "Simasa",
+ "pos_x": 3.28125,
+ "pos_y": -200.28125,
+ "pos_z": 102.1875,
+ "stations": "Shiras Depot,Schroter Landing,MacCurdy Hub,Harvey-Smith Platform"
+ },
+ {
+ "name": "Simte",
+ "pos_x": 65.21875,
+ "pos_y": 58.3125,
+ "pos_z": -94.875,
+ "stations": "Danvers Station,Scott Silo,Kummer Landing,Payton Prospect"
+ },
+ {
+ "name": "Simyr",
+ "pos_x": 81.78125,
+ "pos_y": -19.8125,
+ "pos_z": -95.1875,
+ "stations": "Windt Hub,McArthur Landing,Rothman Terminal,Forward Enterprise"
+ },
+ {
+ "name": "Sinann",
+ "pos_x": -40.65625,
+ "pos_y": 15.875,
+ "pos_z": 64.625,
+ "stations": "Phillips Arena,Jones Point,Spassky Base"
+ },
+ {
+ "name": "Singa",
+ "pos_x": 204.59375,
+ "pos_y": -155.5,
+ "pos_z": 87.71875,
+ "stations": "Leydenfrost Landing,Eggleton Base"
+ },
+ {
+ "name": "Singarli",
+ "pos_x": 55.875,
+ "pos_y": -58.625,
+ "pos_z": 77.1875,
+ "stations": "Abbot Port,Selberg Terminal,Molyneux City,Szameit Relay,Battani Gateway"
+ },
+ {
+ "name": "Singhainau",
+ "pos_x": 30.15625,
+ "pos_y": -217.21875,
+ "pos_z": 66.1875,
+ "stations": "Perlmutter Point"
+ },
+ {
+ "name": "Singu",
+ "pos_x": -67.84375,
+ "pos_y": -46.03125,
+ "pos_z": 87.28125,
+ "stations": "Wilcutt Camp,Atwater Legacy"
+ },
+ {
+ "name": "Sinistra",
+ "pos_x": -47.53125,
+ "pos_y": 16.75,
+ "pos_z": 142.03125,
+ "stations": "Margulis Hub,Napier Oasis"
+ },
+ {
+ "name": "Sint Hozho",
+ "pos_x": -36.8125,
+ "pos_y": 37.90625,
+ "pos_z": -157.03125,
+ "stations": "Leichhardt Survey,Ing Relay,Serrao Landing"
+ },
+ {
+ "name": "Sintangor",
+ "pos_x": -117.65625,
+ "pos_y": 107.78125,
+ "pos_z": -42.0625,
+ "stations": "Piercy Beacon"
+ },
+ {
+ "name": "Sionesta",
+ "pos_x": 50.21875,
+ "pos_y": -24.84375,
+ "pos_z": -40.9375,
+ "stations": "Kubasov Ring,Fawcett Prospect,Volterra Point"
+ },
+ {
+ "name": "Sippi",
+ "pos_x": 135.3125,
+ "pos_y": -118.21875,
+ "pos_z": 15.1875,
+ "stations": "Zaschka Station,Juan de la Cierva Vision,Bothezat Vision"
+ },
+ {
+ "name": "Sippidi",
+ "pos_x": 31.75,
+ "pos_y": -49.96875,
+ "pos_z": 132.90625,
+ "stations": "Islam Terminal,Luyten Orbital,Aller Vision"
+ },
+ {
+ "name": "Siren",
+ "pos_x": 16.125,
+ "pos_y": 22.28125,
+ "pos_z": -77.375,
+ "stations": "Detmer Prospect,Conrad Port,Brash Dock,Wisniewski-Snerg Point,Leestma Beacon"
+ },
+ {
+ "name": "Sireo",
+ "pos_x": 141.125,
+ "pos_y": 12.625,
+ "pos_z": -55.71875,
+ "stations": "Burstein Base,Turtledove Prospect,Dann Port,Lundwall Landing"
+ },
+ {
+ "name": "Siriaba",
+ "pos_x": 89.625,
+ "pos_y": -107.59375,
+ "pos_z": -9.53125,
+ "stations": "Maughmer Refinery,Aoki Dock,Hoyle Refinery,McIntyre Plant,Helin City"
+ },
+ {
+ "name": "Siris",
+ "pos_x": 131.0625,
+ "pos_y": -73.59375,
+ "pos_z": -11.25,
+ "stations": "de Seversky Beacon,Zeppelin's Claim,van de Hulst's Claim"
+ },
+ {
+ "name": "Siristha",
+ "pos_x": 116.75,
+ "pos_y": -176.46875,
+ "pos_z": 11.84375,
+ "stations": "Kepler Enterprise,Coddington Vision,Kirshner Landing,Gann Installation"
+ },
+ {
+ "name": "Sirius",
+ "pos_x": 6.25,
+ "pos_y": -1.28125,
+ "pos_z": -5.75,
+ "stations": "Qwent Research Base,Patterson Enterprise,Efremov Plant,O'Brien Vision"
+ },
+ {
+ "name": "Siro",
+ "pos_x": -103.375,
+ "pos_y": 92.40625,
+ "pos_z": 60.75,
+ "stations": "Garneau Gateway,Morgan Gateway,Leclerc Hub,Crumey Plant"
+ },
+ {
+ "name": "Sirobogama",
+ "pos_x": -122.84375,
+ "pos_y": 87.03125,
+ "pos_z": 62.53125,
+ "stations": "Russ Colony,Pook Dock,Taine Hub,Byrd Installation"
+ },
+ {
+ "name": "Sirona",
+ "pos_x": 75.3125,
+ "pos_y": 66.5,
+ "pos_z": 89.65625,
+ "stations": "Pascal Depot"
+ },
+ {
+ "name": "Sironistula",
+ "pos_x": 29,
+ "pos_y": -73.5625,
+ "pos_z": -114.8125,
+ "stations": "Bouch Landing,Cunningham Settlement,Savitskaya Dock"
+ },
+ {
+ "name": "Sirsir",
+ "pos_x": 105.0625,
+ "pos_y": 3.9375,
+ "pos_z": 7.34375,
+ "stations": "Mattingly Platform,Bunsen's Folly,Ramon Hub,Mitchell Hangar,Dedman Keep"
+ },
+ {
+ "name": "Sisi",
+ "pos_x": 60.46875,
+ "pos_y": 56.40625,
+ "pos_z": -119.875,
+ "stations": "Weston Landing,van Vogt Silo"
+ },
+ {
+ "name": "Sisii",
+ "pos_x": -37.09375,
+ "pos_y": -14.71875,
+ "pos_z": -65.09375,
+ "stations": "Tyson Gateway"
+ },
+ {
+ "name": "Sisina",
+ "pos_x": -54.5625,
+ "pos_y": -98.6875,
+ "pos_z": 77.53125,
+ "stations": "Herndon Stop,Offutt Terminal"
+ },
+ {
+ "name": "Sisini",
+ "pos_x": -1.15625,
+ "pos_y": -24.8125,
+ "pos_z": -101.09375,
+ "stations": "Maxwell Relay"
+ },
+ {
+ "name": "Sitakapa",
+ "pos_x": 83.125,
+ "pos_y": -20.25,
+ "pos_z": 85.03125,
+ "stations": "Kubokawa Terminal,Hanke-Woods Orbital,Celsius Orbital,Russell Hub,Finlay-Freundlich Port,Heinemann Hub,Malvern Dock,Shiner Keep,Bryant Horizons"
+ },
+ {
+ "name": "Sitla",
+ "pos_x": 155.15625,
+ "pos_y": -133.96875,
+ "pos_z": -4.125,
+ "stations": "Stechkin Relay,Bolden Survey"
+ },
+ {
+ "name": "Sitlanei",
+ "pos_x": -64.5625,
+ "pos_y": 53.09375,
+ "pos_z": 101.53125,
+ "stations": "von Bellingshausen Orbital,Lasswitz Beacon,Hill Station,Burton Port,Patterson Point,Tsunenaga Hub,Polya Beacon,Haldeman II Enterprise,Ings Hub,Rasmussen Port,Miklouho-Maclay Port"
+ },
+ {
+ "name": "Siusalk",
+ "pos_x": 66.53125,
+ "pos_y": 6.0625,
+ "pos_z": -148,
+ "stations": "Fife Gateway,Mourelle Orbital"
+ },
+ {
+ "name": "Siusi",
+ "pos_x": 10,
+ "pos_y": -121.125,
+ "pos_z": -112.90625,
+ "stations": "Kimberlin Base,Rothfuss Platform,Cantor Freeport"
+ },
+ {
+ "name": "Sivaci",
+ "pos_x": -121.09375,
+ "pos_y": -41.1875,
+ "pos_z": -44.25,
+ "stations": "Wetherbee Horizons"
+ },
+ {
+ "name": "Sivara",
+ "pos_x": 97.0625,
+ "pos_y": -94.875,
+ "pos_z": 95.90625,
+ "stations": "Suri Port,Spassky Landing,Sunyaev Hub,Bohrmann Orbital,Tennyson d'Eyncourt Vision"
+ },
+ {
+ "name": "Sivas",
+ "pos_x": -69.0625,
+ "pos_y": 42.59375,
+ "pos_z": 38.875,
+ "stations": "Sharp Station,Cavalieri Hub,Thurston Enterprise,Oramus Vista,Laumer Station,Serrao Port,Pierce Gateway,Zamyatin Hub,Archambault Terminal,Matheson Installation"
+ },
+ {
+ "name": "Skadjalini",
+ "pos_x": 67.875,
+ "pos_y": -70.46875,
+ "pos_z": 18.375,
+ "stations": "Matthaus Olbers Enterprise,Olahus Works,McCrea Orbital,Wirtanen Orbital"
+ },
+ {
+ "name": "Skadjero",
+ "pos_x": -138.6875,
+ "pos_y": -58.6875,
+ "pos_z": 28.34375,
+ "stations": "Korniyenko Dock,Tudela Base"
+ },
+ {
+ "name": "Skandini",
+ "pos_x": 165.21875,
+ "pos_y": -17.8125,
+ "pos_z": 27.125,
+ "stations": "Przhevalsky Holdings,Stableford Refinery,Bradbury Installation,Robinson Reach"
+ },
+ {
+ "name": "Skang",
+ "pos_x": -4.09375,
+ "pos_y": 36.46875,
+ "pos_z": -89.4375,
+ "stations": "Behnken Dock,Thompson's Progress,Midgley Settlement"
+ },
+ {
+ "name": "Skaoismont",
+ "pos_x": -103.28125,
+ "pos_y": -56.3125,
+ "pos_z": 19.65625,
+ "stations": "Wingqvist Station"
+ },
+ {
+ "name": "Skappa",
+ "pos_x": -61,
+ "pos_y": -59,
+ "pos_z": -42.3125,
+ "stations": "Moriarty Plant,Beltrami Terminal,Dampier Reach,Fast Refinery"
+ },
+ {
+ "name": "Skardee",
+ "pos_x": 89.46875,
+ "pos_y": -3.5625,
+ "pos_z": -2.625,
+ "stations": "Heck Reserve"
+ },
+ {
+ "name": "Skat",
+ "pos_x": -60.03125,
+ "pos_y": -140.625,
+ "pos_z": 49.15625,
+ "stations": "Chadwick Station,Alden Terminal,Griffith Lab"
+ },
+ {
+ "name": "Skaudai CH-B d14-34",
+ "pos_x": -5481.84375,
+ "pos_y": -579.15625,
+ "pos_z": 10429.9375,
+ "stations": "Sacaqawea Space Port"
+ },
+ {
+ "name": "Skaut",
+ "pos_x": 85.375,
+ "pos_y": 41.90625,
+ "pos_z": 23.0625,
+ "stations": "Taylor Platform,Ziemianski Vision,Elwood Barracks"
+ },
+ {
+ "name": "Skegante",
+ "pos_x": 15.5,
+ "pos_y": -226.0625,
+ "pos_z": 32.84375,
+ "stations": "Struzan Mines,Anderson Bastion,Nijland Dock"
+ },
+ {
+ "name": "Skegasaei",
+ "pos_x": -99.78125,
+ "pos_y": 18.59375,
+ "pos_z": 30.96875,
+ "stations": "H. G. Wells Settlement,Grant Relay,Soto Vision,Swift Station,Wingqvist Keep"
+ },
+ {
+ "name": "Skeggiko O",
+ "pos_x": 53.6875,
+ "pos_y": 11.9375,
+ "pos_z": 16.5,
+ "stations": "Hewish's Inheritance,Kuo Terminal,Crown Horizons,Cabot's Inheritance,Bean Arsenal"
+ },
+ {
+ "name": "Skeller",
+ "pos_x": 3.9375,
+ "pos_y": -90.71875,
+ "pos_z": -35.6875,
+ "stations": "Linge Station,Geston Enterprise,Heck Dock"
+ },
+ {
+ "name": "Skil'sla",
+ "pos_x": -156.75,
+ "pos_y": -23.0625,
+ "pos_z": 76.40625,
+ "stations": "Bates Survey"
+ },
+ {
+ "name": "Skil'slandi",
+ "pos_x": 62.125,
+ "pos_y": -232.28125,
+ "pos_z": 69.5625,
+ "stations": "Plait Hub,Albumasar Port,Kimura Vision"
+ },
+ {
+ "name": "Skinfaxi",
+ "pos_x": -29.3125,
+ "pos_y": -39.625,
+ "pos_z": 34.28125,
+ "stations": "Barnwell Hub,Watts Co-operative,Eilenberg Co-operative,Ziemianski Bastion"
+ },
+ {
+ "name": "Skiraja",
+ "pos_x": 135.65625,
+ "pos_y": 46.09375,
+ "pos_z": -73.15625,
+ "stations": "Wingrove Orbital,Robinson Horizons,Bruce Orbital,Dantec Prospect,Keyes Hub"
+ },
+ {
+ "name": "Skiraragea",
+ "pos_x": 64.15625,
+ "pos_y": -75.53125,
+ "pos_z": 31.375,
+ "stations": "J. G. Ballard Holdings,McMahon Depot,Naubakht Platform,Pu Settlement"
+ },
+ {
+ "name": "Skogulumari",
+ "pos_x": 70.03125,
+ "pos_y": -10,
+ "pos_z": 67.21875,
+ "stations": "Matheson Gateway,Palmer Dock,Nicholson Dock,Aguirre City,Holland Arsenal,Hartlib Arena,Grigson City,Butler Port,Ahnert Installation,Al-Kashi Dock,Lubin Enterprise,Martinez Vista"
+ },
+ {
+ "name": "Skoko",
+ "pos_x": 168.03125,
+ "pos_y": -222.375,
+ "pos_z": 51.53125,
+ "stations": "Butler Landing,Palitzsch Works,Kerimov Works"
+ },
+ {
+ "name": "Skokomish",
+ "pos_x": -116.9375,
+ "pos_y": -70.84375,
+ "pos_z": 4.28125,
+ "stations": "Ballard Penal colony,Sturt Orbital,Whymper Beacon"
+ },
+ {
+ "name": "Skoll",
+ "pos_x": 14.125,
+ "pos_y": -49.78125,
+ "pos_z": 143.4375,
+ "stations": "Humboldt Prospect,Lysenko's Folly"
+ },
+ {
+ "name": "Skollanga",
+ "pos_x": 37.21875,
+ "pos_y": 63.8125,
+ "pos_z": -91.21875,
+ "stations": "Makeev Dock,Fuca Dock,Shipton Dock"
+ },
+ {
+ "name": "Skollobog",
+ "pos_x": -70.875,
+ "pos_y": 34.75,
+ "pos_z": 131.125,
+ "stations": "Payson Legacy"
+ },
+ {
+ "name": "Skollung",
+ "pos_x": -61.15625,
+ "pos_y": -22.4375,
+ "pos_z": -4.6875,
+ "stations": "Melvin Hub,Euler Hub,Ray Ring,Moore Market"
+ },
+ {
+ "name": "Skuta",
+ "pos_x": 38.5,
+ "pos_y": -10.34375,
+ "pos_z": -89.09375,
+ "stations": "Herreshoff Point,Frimout Platform"
+ },
+ {
+ "name": "Slatas",
+ "pos_x": 52.4375,
+ "pos_y": -173.125,
+ "pos_z": 20.8125,
+ "stations": "von Zach Station,Bennett Vision,Rubin Station,Burke Holdings"
+ },
+ {
+ "name": "Slatus",
+ "pos_x": 76.6875,
+ "pos_y": 35.1875,
+ "pos_z": -19.09375,
+ "stations": "Tyson Port,Ham Hub,Hadfield Station,Culbertson Port,Duke Orbital,Sharma Enterprise,Alvares Horizons,Clayton Settlement"
+ },
+ {
+ "name": "Slavaci",
+ "pos_x": -35.71875,
+ "pos_y": -182.625,
+ "pos_z": 41.59375,
+ "stations": "Perlmutter Settlement,Gent Landing,Lerner Holdings"
+ },
+ {
+ "name": "Slavanibo",
+ "pos_x": -87.78125,
+ "pos_y": 24.65625,
+ "pos_z": 26.1875,
+ "stations": "Irwin Mines,Gibson Installation,Melnick Works"
+ },
+ {
+ "name": "Slavisc",
+ "pos_x": 40.6875,
+ "pos_y": -99.96875,
+ "pos_z": -17.09375,
+ "stations": "Farmer Prospect,Siodmak Dock,Wallis Mine"
+ },
+ {
+ "name": "Sleipnera",
+ "pos_x": -184.125,
+ "pos_y": 19.21875,
+ "pos_z": -6.75,
+ "stations": "Byrd Outpost,Alvarez de Pineda's Exile,Caryanda Plant"
+ },
+ {
+ "name": "Sleipnir",
+ "pos_x": 158.53125,
+ "pos_y": -21.375,
+ "pos_z": 20.40625,
+ "stations": "Bowell Vision,Kirshner Plant,Malcolm Arena"
+ },
+ {
+ "name": "Sleza Viron",
+ "pos_x": 121.75,
+ "pos_y": -52.28125,
+ "pos_z": 126.5625,
+ "stations": "Harrington Prospect,Pu Station,Schmidt Bastion"
+ },
+ {
+ "name": "Slink's Eye",
+ "pos_x": -10.125,
+ "pos_y": -29.6875,
+ "pos_z": -10.15625,
+ "stations": "Kelleam Ring,Beckman Prospect"
+ },
+ {
+ "name": "Smei Ti",
+ "pos_x": 119.59375,
+ "pos_y": 4.5,
+ "pos_z": -27.3125,
+ "stations": "Dalton Refinery,Popov Vision"
+ },
+ {
+ "name": "Smei Tsu",
+ "pos_x": -30.90625,
+ "pos_y": -104.6875,
+ "pos_z": 62,
+ "stations": "Banneker Installation,Shajn City,Mitchell Observatory,Gora Installation,Meinel Installation"
+ },
+ {
+ "name": "Smeits",
+ "pos_x": -2.5625,
+ "pos_y": -198.90625,
+ "pos_z": 64,
+ "stations": "Yamamoto Relay,Geller Mines"
+ },
+ {
+ "name": "Smethells 1",
+ "pos_x": 49.875,
+ "pos_y": -20.71875,
+ "pos_z": 71.40625,
+ "stations": "Kadenyuk Refinery,Heng Relay"
+ },
+ {
+ "name": "Smethells 102",
+ "pos_x": 3.90625,
+ "pos_y": -92.90625,
+ "pos_z": 66.25,
+ "stations": "Lange Base,Borrego Arena"
+ },
+ {
+ "name": "Smethells 119",
+ "pos_x": 18.03125,
+ "pos_y": -68.0625,
+ "pos_z": 39.71875,
+ "stations": "Roberts Terminal,Baily Barracks,Napier Arena,Cherry's Inheritance,Godwin Survey,Thoreau Refinery"
+ },
+ {
+ "name": "Smethells 173",
+ "pos_x": 44.59375,
+ "pos_y": -120.03125,
+ "pos_z": 30.46875,
+ "stations": "Babcock Port,Schumacher Station,Antonov Survey"
+ },
+ {
+ "name": "Smethells 56",
+ "pos_x": 11.53125,
+ "pos_y": -64.4375,
+ "pos_z": 85,
+ "stations": "Aldiss Beacon,Williams Holdings,Moisuc Dock,Elder Base,Carr Orbital"
+ },
+ {
+ "name": "Smethells 97",
+ "pos_x": 8.96875,
+ "pos_y": -73.84375,
+ "pos_z": 57.78125,
+ "stations": "Rasmussen Enterprise,Cousin Hub,Mitropoulos Ring,Heceta Barracks,Jones Vision"
+ },
+ {
+ "name": "Smolyadaker",
+ "pos_x": 99.46875,
+ "pos_y": -3.5625,
+ "pos_z": -92.65625,
+ "stations": "Williams Platform,Phillifent Mine,Roddenberry Point"
+ },
+ {
+ "name": "Smolyaqa",
+ "pos_x": -35.3125,
+ "pos_y": -79.96875,
+ "pos_z": 46.5625,
+ "stations": "Leberecht Tempel Terminal,Donaldson's Inheritance,Gehrels Orbital,Huxley Works"
+ },
+ {
+ "name": "Snoqui",
+ "pos_x": -138.21875,
+ "pos_y": -58.625,
+ "pos_z": -33.90625,
+ "stations": "Fossey Survey"
+ },
+ {
+ "name": "Snoqui Xian",
+ "pos_x": -73.78125,
+ "pos_y": -28.375,
+ "pos_z": -36.59375,
+ "stations": "Conrad Vision,Cixin Base,Brahe Relay"
+ },
+ {
+ "name": "Snoquot",
+ "pos_x": -34.21875,
+ "pos_y": -63.40625,
+ "pos_z": -60.21875,
+ "stations": "Wrangell City,Hooke Enterprise,Horowitz Dock,Montrose Silo,Selberg Landing"
+ },
+ {
+ "name": "Snotock Ek",
+ "pos_x": 59.09375,
+ "pos_y": -155.84375,
+ "pos_z": 96.3125,
+ "stations": "Cowling Survey,Gabrielli Platform,Anthony Barracks,Schwarzschild Platform"
+ },
+ {
+ "name": "Snotricopa",
+ "pos_x": 31.5,
+ "pos_y": -12.6875,
+ "pos_z": -90.46875,
+ "stations": "Meitner Gateway,Hobaugh Settlement,vo Ring,Kroehl Terminal,Hovgaard Depot"
+ },
+ {
+ "name": "Sobek",
+ "pos_x": -34.46875,
+ "pos_y": 67.78125,
+ "pos_z": -21.09375,
+ "stations": "Landis Freeport"
+ },
+ {
+ "name": "Soch",
+ "pos_x": 93.125,
+ "pos_y": -60.6875,
+ "pos_z": 23.40625,
+ "stations": "Whitcomb Ring,Baxter Silo,Marriott Escape,Seamans Vision"
+ },
+ {
+ "name": "Sochet",
+ "pos_x": 126.34375,
+ "pos_y": 0.125,
+ "pos_z": 101.03125,
+ "stations": "Yolen's Progress"
+ },
+ {
+ "name": "Socho",
+ "pos_x": -72.4375,
+ "pos_y": -101.15625,
+ "pos_z": -141.1875,
+ "stations": "Chwedyk Orbital,Malchiodi Dock,Dantec Enterprise"
+ },
+ {
+ "name": "Sofagre",
+ "pos_x": -54.21875,
+ "pos_y": 48.53125,
+ "pos_z": -30.15625,
+ "stations": "North's Claim,al-Khowarizmi Landing,Elwood Dock"
+ },
+ {
+ "name": "Soholia",
+ "pos_x": -11.5,
+ "pos_y": 96.3125,
+ "pos_z": -21.96875,
+ "stations": "Berlin,Kyoto Park,Julian Terminal,Ford Depot,Nikitin Settlement"
+ },
+ {
+ "name": "Sokandjiru",
+ "pos_x": 66.71875,
+ "pos_y": 128.3125,
+ "pos_z": -5.96875,
+ "stations": "Oppenheimer Terminal"
+ },
+ {
+ "name": "Sokara",
+ "pos_x": -105.21875,
+ "pos_y": -65.15625,
+ "pos_z": 83.34375,
+ "stations": "Musa al-Khwarizmi Station,Nixon Relay,McDaniel Hub"
+ },
+ {
+ "name": "Sokaram",
+ "pos_x": 151.09375,
+ "pos_y": -193.09375,
+ "pos_z": 89.78125,
+ "stations": "Porco Gateway,Biermann Vision,van der Riet Woolley Vision,Lee Legacy,Nelder Base"
+ },
+ {
+ "name": "Sokariang",
+ "pos_x": 116.5,
+ "pos_y": -101.28125,
+ "pos_z": 58.28125,
+ "stations": "Hulse Hub,Penzias City,Halley Vision,McIntyre Horizons,Mueller Keep,Rankin Hub,Mechain Enterprise"
+ },
+ {
+ "name": "Sokat",
+ "pos_x": 121.90625,
+ "pos_y": -215.53125,
+ "pos_z": 34.28125,
+ "stations": "Gutierrez Hub,Battani Orbital"
+ },
+ {
+ "name": "Sokate",
+ "pos_x": 117.1875,
+ "pos_y": -103.03125,
+ "pos_z": 98.8125,
+ "stations": "Trumpler Dock,Behnisch Terminal,Bartini Stop,Bradfield Relay"
+ },
+ {
+ "name": "Sokathani",
+ "pos_x": 200.0625,
+ "pos_y": -168.46875,
+ "pos_z": -14.78125,
+ "stations": "Ban Terminal,Fallows' Progress"
+ },
+ {
+ "name": "Sokatines",
+ "pos_x": 60.9375,
+ "pos_y": -126.25,
+ "pos_z": 117.34375,
+ "stations": "Inoda Ring"
+ },
+ {
+ "name": "Sokn",
+ "pos_x": -51.96875,
+ "pos_y": -79.0625,
+ "pos_z": -11.34375,
+ "stations": "Whitelaw Station"
+ },
+ {
+ "name": "Soko Oh",
+ "pos_x": -98,
+ "pos_y": 75.09375,
+ "pos_z": -57.84375,
+ "stations": "Dyson Vision,Hooke Point"
+ },
+ {
+ "name": "Sokobii",
+ "pos_x": -50.625,
+ "pos_y": -152.71875,
+ "pos_z": 27.71875,
+ "stations": "Dashiell Orbital,Pelt Orbital,Stiegler Station,Foster Penal colony"
+ },
+ {
+ "name": "Sokoi",
+ "pos_x": 142.53125,
+ "pos_y": -150.5625,
+ "pos_z": -24.9375,
+ "stations": "Cowell Hub,Kopal Survey"
+ },
+ {
+ "name": "Sokoji",
+ "pos_x": 58.15625,
+ "pos_y": 38.875,
+ "pos_z": 19.4375,
+ "stations": "Ellis Refinery,White's Progress,Locke Prospect"
+ },
+ {
+ "name": "Sokojiu",
+ "pos_x": 122.34375,
+ "pos_y": 12.75,
+ "pos_z": -80.21875,
+ "stations": "Lopez de Legazpi Station,Stasheff Terminal,Rucker Enterprise,McMullen Enterprise"
+ },
+ {
+ "name": "Sokolongo",
+ "pos_x": 142.84375,
+ "pos_y": -93.28125,
+ "pos_z": -77.78125,
+ "stations": "Ambartsumian Works,Archer Installation"
+ },
+ {
+ "name": "Sokolsci",
+ "pos_x": 64.78125,
+ "pos_y": -61.75,
+ "pos_z": -62.21875,
+ "stations": "Manarov Vision,Chaudhary Orbital,Holberg Survey"
+ },
+ {
+ "name": "Sokomi",
+ "pos_x": 41.3125,
+ "pos_y": -163.5,
+ "pos_z": 131.0625,
+ "stations": "Zeppelin Dock,Robert Port,Sy Orbital,Rond d'Alembert's Inheritance,Bombelli Prospect"
+ },
+ {
+ "name": "Sokond",
+ "pos_x": 6.4375,
+ "pos_y": -64.78125,
+ "pos_z": 161.875,
+ "stations": "Tanner Ring,Meade Colony,Blaha Terminal"
+ },
+ {
+ "name": "Sokopel",
+ "pos_x": 96.90625,
+ "pos_y": 23.09375,
+ "pos_z": 122.09375,
+ "stations": "Desargues Base,Pierce Barracks,Bursch Terminal"
+ },
+ {
+ "name": "Sokoq",
+ "pos_x": -18.6875,
+ "pos_y": 37.15625,
+ "pos_z": 80.28125,
+ "stations": "Andrews Stop"
+ },
+ {
+ "name": "Sol",
+ "pos_x": 0,
+ "pos_y": 0,
+ "pos_z": 0,
+ "stations": "Columbus,Dekker's Yard,Abraham Lincoln,Daedalus,Galileo,M.Gorbachev,Titan City,Li Qing Jao,Mars High,Ehrlich City,Walz Depot,Burnell Station,Durrance Camp,Furukawa Enterprise,Haberlandt Survey,Schottky Reformatory"
+ },
+ {
+ "name": "Solati",
+ "pos_x": 66.53125,
+ "pos_y": 29.1875,
+ "pos_z": 34.6875,
+ "stations": "ELWireCraft,Solati Reach,Hawking Gateway,Ising Refinery,McCandless Base"
+ },
+ {
+ "name": "Solibamba",
+ "pos_x": 99.5625,
+ "pos_y": 40.125,
+ "pos_z": 26.8125,
+ "stations": "Vetulani Dock,Butz Mines"
+ },
+ {
+ "name": "Solitude",
+ "pos_x": -9497.65625,
+ "pos_y": -911,
+ "pos_z": 19807.625,
+ "stations": "Knight's Retreat"
+ },
+ {
+ "name": "Sollaro",
+ "pos_x": -9528.625,
+ "pos_y": -885.59375,
+ "pos_z": 19815.4375,
+ "stations": "Annan Orbital"
+ },
+ {
+ "name": "Soma",
+ "pos_x": -94.4375,
+ "pos_y": -15.3125,
+ "pos_z": -2.46875,
+ "stations": "Cremona Reach,Michelson Orbital,Winne Orbital"
+ },
+ {
+ "name": "Somtupi",
+ "pos_x": -29.25,
+ "pos_y": -159.78125,
+ "pos_z": -43.375,
+ "stations": "Quetelet Reformatory"
+ },
+ {
+ "name": "Song Ku",
+ "pos_x": -148.4375,
+ "pos_y": 28.5625,
+ "pos_z": -5.40625,
+ "stations": "Robinson Station,Arthur Terminal,Baker Enterprise,Stephens Depot"
+ },
+ {
+ "name": "Song Tzu",
+ "pos_x": 171.9375,
+ "pos_y": 43.46875,
+ "pos_z": -35.5,
+ "stations": "Silverberg Stop,Wnuk-Lipinski Installation"
+ },
+ {
+ "name": "Songa",
+ "pos_x": -63.8125,
+ "pos_y": -139.0625,
+ "pos_z": 124.5,
+ "stations": "Merril Hub,Witt Landing,Phillips Works,Robinson Settlement,Robinson Hub"
+ },
+ {
+ "name": "Songala",
+ "pos_x": -150.25,
+ "pos_y": -21.65625,
+ "pos_z": 79.53125,
+ "stations": "Lee's Claim,Rocklynne Depot,Fernandes de Queiros Colony"
+ },
+ {
+ "name": "Songballa",
+ "pos_x": 32.375,
+ "pos_y": -117.75,
+ "pos_z": -81,
+ "stations": "Herbert Dock"
+ },
+ {
+ "name": "Songbe",
+ "pos_x": -19.84375,
+ "pos_y": -129.5625,
+ "pos_z": -27.40625,
+ "stations": "Precourt City,du Fresne Survey,Jun Dock,Cockrell Landing,Fuglesang Station"
+ },
+ {
+ "name": "Songi",
+ "pos_x": 100.15625,
+ "pos_y": -17.09375,
+ "pos_z": 63.71875,
+ "stations": "Brosnan Prospect,Mayr Keep"
+ },
+ {
+ "name": "Songlalis",
+ "pos_x": -68.65625,
+ "pos_y": 51.15625,
+ "pos_z": -72.25,
+ "stations": "Fernandez Gateway,Salgari Station,Reed Barracks"
+ },
+ {
+ "name": "Songloi",
+ "pos_x": 23.875,
+ "pos_y": -165.84375,
+ "pos_z": -21.6875,
+ "stations": "Pickering Point,Gallun Survey,Dyson Terminal"
+ },
+ {
+ "name": "Songo",
+ "pos_x": 160.5,
+ "pos_y": -206.3125,
+ "pos_z": 26.90625,
+ "stations": "Chadbourne Orbital,Pittendreigh Port,Reinmuth Depot"
+ },
+ {
+ "name": "Songol",
+ "pos_x": -1.78125,
+ "pos_y": 70.59375,
+ "pos_z": -53.78125,
+ "stations": "Cernan Station,Glazkov Gateway,Lloyd Point,Goddard Enterprise,Napier Prospect"
+ },
+ {
+ "name": "Songye",
+ "pos_x": 122.28125,
+ "pos_y": -115.90625,
+ "pos_z": -80.53125,
+ "stations": "Zarnecki Settlement"
+ },
+ {
+ "name": "Songzi",
+ "pos_x": 95,
+ "pos_y": -161.875,
+ "pos_z": -4.71875,
+ "stations": "van der Riet Woolley Vision,McIntosh Terminal,Alexandria Gateway,Piccard Prospect"
+ },
+ {
+ "name": "Soniteru",
+ "pos_x": 41.6875,
+ "pos_y": -161.75,
+ "pos_z": -24.125,
+ "stations": "Giacconi Platform,LeConte Installation"
+ },
+ {
+ "name": "Sons",
+ "pos_x": 1.28125,
+ "pos_y": 78.78125,
+ "pos_z": -59.1875,
+ "stations": "Soukup Hub,Ross Enterprise,Alvarado City"
+ },
+ {
+ "name": "Sopdet",
+ "pos_x": 103.90625,
+ "pos_y": -140.28125,
+ "pos_z": 57.40625,
+ "stations": "Fujikawa Dock,Al Sufi's Claim,Stechkin Platform,Iwamoto Port"
+ },
+ {
+ "name": "Sopdu",
+ "pos_x": -45.625,
+ "pos_y": 7.125,
+ "pos_z": -27.53125,
+ "stations": "Arthur Keep,McNair Refinery,Thiele's Progress,Jendrassik Orbital"
+ },
+ {
+ "name": "Sopedu",
+ "pos_x": 0.40625,
+ "pos_y": -224.46875,
+ "pos_z": 40,
+ "stations": "Bok Enterprise,Abel Landing,Birkhoff Settlement,Chern Arsenal"
+ },
+ {
+ "name": "Sopema",
+ "pos_x": -99.71875,
+ "pos_y": -124.0625,
+ "pos_z": -40.28125,
+ "stations": "Hyecho Observatory,Hennepin Platform"
+ },
+ {
+ "name": "Sopo Vuh",
+ "pos_x": 162.25,
+ "pos_y": -78.84375,
+ "pos_z": 53.40625,
+ "stations": "Maler Base,Beshore Port,Yerka Dock"
+ },
+ {
+ "name": "Soponna",
+ "pos_x": -114.96875,
+ "pos_y": -30.3125,
+ "pos_z": 36.78125,
+ "stations": "Ramon Settlement"
+ },
+ {
+ "name": "Sopontet",
+ "pos_x": -60.1875,
+ "pos_y": -72.09375,
+ "pos_z": 35.9375,
+ "stations": "Thoreau Port,Parker City,Anning Gateway,Burnet Enterprise,Gernhardt Dock,Cormack City,McDivitt Port,Camarda Terminal,Barentsz Vision,Lerner Terminal"
+ },
+ {
+ "name": "Sorbacoc",
+ "pos_x": 151.0625,
+ "pos_y": -164,
+ "pos_z": 102.6875,
+ "stations": "Izumikawa Port,Corte-Real Oasis,Cavendish Hub"
+ },
+ {
+ "name": "Sorbago",
+ "pos_x": 60.96875,
+ "pos_y": -72.09375,
+ "pos_z": 37.40625,
+ "stations": "Hencke Orbital,Dowie Depot,Dalgarno Colony,Carroll Survey"
+ },
+ {
+ "name": "Sorbs",
+ "pos_x": 45.71875,
+ "pos_y": 67.46875,
+ "pos_z": 121.5625,
+ "stations": "Chu Point,Frechet's Exile"
+ },
+ {
+ "name": "Sosoling",
+ "pos_x": 43.15625,
+ "pos_y": -19.46875,
+ "pos_z": 42.375,
+ "stations": "Ramsay Hub"
+ },
+ {
+ "name": "Sosolingati",
+ "pos_x": 21.3125,
+ "pos_y": -13.875,
+ "pos_z": -2.09375,
+ "stations": "Dana Hub,Murray Refinery,Vlamingh Oasis,Quimby Horizons"
+ },
+ {
+ "name": "Sosong",
+ "pos_x": 73.65625,
+ "pos_y": 7.71875,
+ "pos_z": 18.125,
+ "stations": "Dzhanibekov Station,Dalton Station,Potter Terminal,Chu Orbital,Slayton Terminal,Galilei Platform,Boltzmann Station,Pullman Silo,Sternberg Settlement,Soddy Gateway,VanderMeer Base"
+ },
+ {
+ "name": "Sother",
+ "pos_x": 35.5,
+ "pos_y": 4.78125,
+ "pos_z": -108.59375,
+ "stations": "Baxter Gateway,Asher Penal colony"
+ },
+ {
+ "name": "Sothis",
+ "pos_x": -352.78125,
+ "pos_y": 10.5,
+ "pos_z": -346.34375,
+ "stations": "Newholm Station,Sothis Mining,Don's Inheritance,Velinski Enterprise,Vantage Point"
+ },
+ {
+ "name": "Sotho",
+ "pos_x": 19.125,
+ "pos_y": -117.8125,
+ "pos_z": 166.625,
+ "stations": "Peters Stop,Sutter Outpost"
+ },
+ {
+ "name": "Sotiate",
+ "pos_x": 145.34375,
+ "pos_y": -195.125,
+ "pos_z": 94.53125,
+ "stations": "Sweet Dock,Nagata Mines,Mozhaysky Prospect,Xuanzang Landing"
+ },
+ {
+ "name": "Sotidji",
+ "pos_x": -51.59375,
+ "pos_y": 73.875,
+ "pos_z": 73.15625,
+ "stations": "Kondakova Platform,Yamazaki Orbital"
+ },
+ {
+ "name": "Sotigur",
+ "pos_x": -38.59375,
+ "pos_y": -9.71875,
+ "pos_z": 109.78125,
+ "stations": "Okorafor Bastion,Lorenz Works,Berliner Settlement"
+ },
+ {
+ "name": "Sotigurlu",
+ "pos_x": 61.78125,
+ "pos_y": 100.9375,
+ "pos_z": 9.3125,
+ "stations": "Wakata Survey,Garden Landing,Boulle's Progress"
+ },
+ {
+ "name": "Sotini",
+ "pos_x": -14.53125,
+ "pos_y": -87.34375,
+ "pos_z": 81.40625,
+ "stations": "Gagarin Beacon,Harrison Terminal,Effinger Beacon"
+ },
+ {
+ "name": "Sotuki",
+ "pos_x": 10.6875,
+ "pos_y": 43.0625,
+ "pos_z": -81.15625,
+ "stations": "Wolf Station,Smeaton Market"
+ },
+ {
+ "name": "Sotuknates",
+ "pos_x": -59.625,
+ "pos_y": -161,
+ "pos_z": -22.125,
+ "stations": "Wild Hub,Dillon Platform,Ayerdhal Terminal,Whymper Survey"
+ },
+ {
+ "name": "Soturicari",
+ "pos_x": -34.78125,
+ "pos_y": -34.125,
+ "pos_z": -3.15625,
+ "stations": "Bordage Arena"
+ },
+ {
+ "name": "Sotzil",
+ "pos_x": 120.34375,
+ "pos_y": 71.96875,
+ "pos_z": 118.625,
+ "stations": "Poindexter Enterprise,Bushnell Works"
+ },
+ {
+ "name": "Soul Sector EL-Y d7",
+ "pos_x": -5043.15625,
+ "pos_y": 85.03125,
+ "pos_z": -5513.09375,
+ "stations": "Base Camp"
+ },
+ {
+ "name": "Sounti",
+ "pos_x": 100.15625,
+ "pos_y": 6.625,
+ "pos_z": 17.3125,
+ "stations": "Teng Station,Zindell Station,Reamy Dock,Alten Gateway,Cartier Gateway,Andrews Depot,Bruce Installation"
+ },
+ {
+ "name": "Sovereign's Reach",
+ "pos_x": -9535.96875,
+ "pos_y": -913.78125,
+ "pos_z": 19816.96875,
+ "stations": "Harold's Respite"
+ },
+ {
+ "name": "Sowathara",
+ "pos_x": 78.3125,
+ "pos_y": -144.1875,
+ "pos_z": 8.96875,
+ "stations": "Milne Orbital,Offutt Colony,Van Scyoc Port"
+ },
+ {
+ "name": "Sowiio",
+ "pos_x": 56.4375,
+ "pos_y": -74.03125,
+ "pos_z": 22.375,
+ "stations": "Shaw Port,Bigourdan Ring,Cassini Hub,Wegener Orbital,Janszoon Prospect,Gessi Keep,Morgan Hub,Mooz Ring,Montrose Terminal,Cartmill's Folly,Reamy Landing,Ziegel's Claim,Reeves Prospect"
+ },
+ {
+ "name": "Soyota",
+ "pos_x": 105.875,
+ "pos_y": 86.40625,
+ "pos_z": -59.9375,
+ "stations": "Gohar Hub,Adams Gateway,Hoshide Landing,Blaylock Hub,Bertin Base"
+ },
+ {
+ "name": "SPF-LF 1",
+ "pos_x": 2.90625,
+ "pos_y": 6.3125,
+ "pos_z": -9.5625,
+ "stations": "The Pillar of Fortitude"
+ },
+ {
+ "name": "Spinaje",
+ "pos_x": 89.875,
+ "pos_y": -75.21875,
+ "pos_z": 150.9375,
+ "stations": "Wirtanen Terminal,Ilyushin Station,Suri Vision"
+ },
+ {
+ "name": "Spinibo",
+ "pos_x": 50.96875,
+ "pos_y": -37.875,
+ "pos_z": 43.96875,
+ "stations": "Messerschmid Terminal"
+ },
+ {
+ "name": "Spinoni",
+ "pos_x": -39.65625,
+ "pos_y": -65.75,
+ "pos_z": 9.625,
+ "stations": "Lysenko Barracks,Irwin City"
+ },
+ {
+ "name": "SPOCS 103",
+ "pos_x": -24.34375,
+ "pos_y": -103.4375,
+ "pos_z": -55.21875,
+ "stations": "Furukawa City,Minkowski Base,Strzelecki Prospect"
+ },
+ {
+ "name": "SPOCS 111",
+ "pos_x": -55.875,
+ "pos_y": -64,
+ "pos_z": -76.0625,
+ "stations": "Lysenko Colony"
+ },
+ {
+ "name": "SPOCS 208",
+ "pos_x": 10.8125,
+ "pos_y": -55.40625,
+ "pos_z": -98.71875,
+ "stations": "Napier Prospect,Wakata Orbital,Haise Orbital,Thomas Hub,Burbank Orbital,Kelly City,Morgan Dock,Popov Terminal,Bernoulli Port,Al-Battani Hub,Springer Colony"
+ },
+ {
+ "name": "SPOCS 253",
+ "pos_x": -15.375,
+ "pos_y": -0.625,
+ "pos_z": -83.8125,
+ "stations": "Gauss Orbital,Blish Point,Sturckow Port,Mitropoulos Observatory,Tognini Port,Hermaszewski Station,Laplace Dock,Magnus Dock,Sagan Station,Rescue Ship - Sturckow Port"
+ },
+ {
+ "name": "SPOCS 343",
+ "pos_x": 99.4375,
+ "pos_y": -12.375,
+ "pos_z": -64.03125,
+ "stations": "Galiano Dock"
+ },
+ {
+ "name": "SPOCS 399",
+ "pos_x": 69.09375,
+ "pos_y": 54.0625,
+ "pos_z": -79.5625,
+ "stations": "Santarem Depot,Artin Platform,Santos Relay"
+ },
+ {
+ "name": "SPOCS 419",
+ "pos_x": 60.125,
+ "pos_y": 50.375,
+ "pos_z": -47.65625,
+ "stations": "Nelson Enterprise,Skripochka Hub"
+ },
+ {
+ "name": "SPOCS 486",
+ "pos_x": 87.46875,
+ "pos_y": 69.5625,
+ "pos_z": 9.1875,
+ "stations": "Slade Enterprise,Fernandes de Queiros Gateway,Oliver Relay,Lee Orbital,Alexander Arsenal"
+ },
+ {
+ "name": "SPOCS 495",
+ "pos_x": -9.8125,
+ "pos_y": 107.65625,
+ "pos_z": -40.4375,
+ "stations": "Tapinas Port,Russell's Claim"
+ },
+ {
+ "name": "SPOCS 507",
+ "pos_x": 20.59375,
+ "pos_y": 114.59375,
+ "pos_z": -21.15625,
+ "stations": "Bentham Orbital,Bernoulli's Progress"
+ },
+ {
+ "name": "SPOCS 615",
+ "pos_x": 80.84375,
+ "pos_y": -18.03125,
+ "pos_z": 74.75,
+ "stations": "Larbalestier Station,Tenn Gateway,Rorschach Prospect,Conway Depot,Forward Orbital,Belyanin Relay"
+ },
+ {
+ "name": "SPOCS 678",
+ "pos_x": 64.53125,
+ "pos_y": -11.125,
+ "pos_z": 90.4375,
+ "stations": "Low Camp"
+ },
+ {
+ "name": "SPOCS 681",
+ "pos_x": 28.9375,
+ "pos_y": 34.625,
+ "pos_z": 109.625,
+ "stations": "Bartlett Orbital"
+ },
+ {
+ "name": "SPOCS 719",
+ "pos_x": -70.625,
+ "pos_y": 62.34375,
+ "pos_z": 16.65625,
+ "stations": "Pierres Platform,Jenkinson Survey,Blish Relay"
+ },
+ {
+ "name": "SPOCS 895",
+ "pos_x": -85.21875,
+ "pos_y": -40.53125,
+ "pos_z": 73.40625,
+ "stations": "Maclaurin Colony,Robinson Mines,Cayley's Inheritance,Teller Base"
+ },
+ {
+ "name": "SPOCS 900",
+ "pos_x": 15.21875,
+ "pos_y": -70.21875,
+ "pos_z": 88.40625,
+ "stations": "Bacon Enterprise,Celsius Market,Ricardo Station,Banach Legacy,Parsons Enterprise,Murdoch Enterprise,Whitelaw Prospect"
+ },
+ {
+ "name": "Spokare",
+ "pos_x": 129.3125,
+ "pos_y": 19.375,
+ "pos_z": 60.71875,
+ "stations": "Merchiston Dock,Nicollet Dock"
+ },
+ {
+ "name": "Srnja",
+ "pos_x": -39.78125,
+ "pos_y": 98.1875,
+ "pos_z": -121.625,
+ "stations": "Romanenko Colony"
+ },
+ {
+ "name": "Srnjaki",
+ "pos_x": 29.96875,
+ "pos_y": 83.21875,
+ "pos_z": 64.625,
+ "stations": "Virtanen Enterprise,Matteucci Station,Dowling Beacon,Mendel City,Schlegel Point"
+ },
+ {
+ "name": "SRS 2543",
+ "pos_x": -31.09375,
+ "pos_y": -62.03125,
+ "pos_z": 53.71875,
+ "stations": "Dirac Terminal"
+ },
+ {
+ "name": "Ssu Ling",
+ "pos_x": -76.59375,
+ "pos_y": -57.4375,
+ "pos_z": -87.375,
+ "stations": "Williamson Stop,Goulart Platform"
+ },
+ {
+ "name": "Stafkarl",
+ "pos_x": -17.34375,
+ "pos_y": -88.65625,
+ "pos_z": -33.34375,
+ "stations": "Jaufurally Orbital,Oleskiw Port,Beekman Barracks"
+ },
+ {
+ "name": "Stein 2051",
+ "pos_x": -9.46875,
+ "pos_y": 2.4375,
+ "pos_z": -15.375,
+ "stations": "Boming Station,Rennie Landing"
+ },
+ {
+ "name": "STF 1774",
+ "pos_x": -34.09375,
+ "pos_y": 74.8125,
+ "pos_z": -8.21875,
+ "stations": "Hill Hub,Gabriel Dock,Vizcaino City,Tenn Hub,Coulomb Dock,Brackett Station,Gerrold City,Froude Ring,Henson Bastion,Chalker Enterprise,Hunt Terminal"
+ },
+ {
+ "name": "STF 2841",
+ "pos_x": -78.78125,
+ "pos_y": -40.78125,
+ "pos_z": 19.15625,
+ "stations": "Hackworth Orbital"
+ },
+ {
+ "name": "StHA 181",
+ "pos_x": -38.375,
+ "pos_y": -35.8125,
+ "pos_z": 52.34375,
+ "stations": "Andreas Hub,Wolfe Laboratory"
+ },
+ {
+ "name": "StKM 1-1118",
+ "pos_x": -10.34375,
+ "pos_y": 113.65625,
+ "pos_z": 36.28125,
+ "stations": "Dupuy de Lome Station,Shapiro Enterprise,Shelley Enterprise"
+ },
+ {
+ "name": "StKM 1-1341",
+ "pos_x": -23.09375,
+ "pos_y": 51.84375,
+ "pos_z": 81.5625,
+ "stations": "Horch Survey,Onizuka Oasis,Springer Escape,Berezin Stop"
+ },
+ {
+ "name": "StKM 1-1767",
+ "pos_x": -81.4375,
+ "pos_y": -35.09375,
+ "pos_z": 79.34375,
+ "stations": "Moore Relay,Xing Outpost,Zillig Penal colony"
+ },
+ {
+ "name": "StKM 1-2166",
+ "pos_x": -50.3125,
+ "pos_y": -63.8125,
+ "pos_z": -6.96875,
+ "stations": "Haignere Orbital,Serling Beacon"
+ },
+ {
+ "name": "StKM 1-383",
+ "pos_x": -3.0625,
+ "pos_y": -60.71875,
+ "pos_z": -80.03125,
+ "stations": "Linnaeus Landing,Normand Prospect"
+ },
+ {
+ "name": "StKM 1-442",
+ "pos_x": 31.96875,
+ "pos_y": -55.6875,
+ "pos_z": -40.40625,
+ "stations": "Leavitt Orbital,Toll Relay"
+ },
+ {
+ "name": "StKM 1-478",
+ "pos_x": -0.5625,
+ "pos_y": -50.21875,
+ "pos_z": -108.375,
+ "stations": "Smith Arsenal,Carpini Refinery,Grzimek Installation"
+ },
+ {
+ "name": "StKM 1-578",
+ "pos_x": 32.375,
+ "pos_y": -19.125,
+ "pos_z": -67.96875,
+ "stations": "Allen Survey,Camarda Dock,Manning Beacon,Fung Gateway"
+ },
+ {
+ "name": "StKM 1-616",
+ "pos_x": -27.40625,
+ "pos_y": 32.03125,
+ "pos_z": -63.3125,
+ "stations": "Davy Gateway,Wiberg Hub,Bykovsky Port,Farouk Base"
+ },
+ {
+ "name": "StKM 1-626",
+ "pos_x": 8.6875,
+ "pos_y": 23.09375,
+ "pos_z": -75.65625,
+ "stations": "Dzhanibekov Orbital,Fleming City,Worden Port,Rorschach Orbital,Fujimori Terminal"
+ },
+ {
+ "name": "StKM 1-662",
+ "pos_x": 53.53125,
+ "pos_y": 20.90625,
+ "pos_z": -69.53125,
+ "stations": "Reed Point,Haxel Base,Wundt Station"
+ },
+ {
+ "name": "StKM 1-823",
+ "pos_x": 44.40625,
+ "pos_y": 54.75,
+ "pos_z": -32.53125,
+ "stations": "Shinn Relay"
+ },
+ {
+ "name": "Stopover",
+ "pos_x": -1.65625,
+ "pos_y": -10.28125,
+ "pos_z": 16.625,
+ "stations": "Darkwater Station"
+ },
+ {
+ "name": "Strian",
+ "pos_x": 145.5625,
+ "pos_y": -78.125,
+ "pos_z": 103.75,
+ "stations": "Chebyshev Prospect,Darnielle Works,Husband Station,Common Estate"
+ },
+ {
+ "name": "Strianji",
+ "pos_x": 24.625,
+ "pos_y": -95.65625,
+ "pos_z": 50.03125,
+ "stations": "Belyavsky Station,Lloyd Wright Hub,Charlois Town,Schaumasse Vision"
+ },
+ {
+ "name": "Stribes",
+ "pos_x": 115.25,
+ "pos_y": -98.46875,
+ "pos_z": -60.21875,
+ "stations": "Kirshner Landing"
+ },
+ {
+ "name": "Strigenses",
+ "pos_x": 0.03125,
+ "pos_y": 17.5,
+ "pos_z": -122.65625,
+ "stations": "Low Depot,Boas Horizons"
+ },
+ {
+ "name": "Struve 1321",
+ "pos_x": -14.46875,
+ "pos_y": -3.09375,
+ "pos_z": -13.65625,
+ "stations": "Leslie Town,Thiele Station,Levi-Strauss City,Apt Station,Emshwiller Enterprise"
+ },
+ {
+ "name": "Stuelou AT-J c25-24",
+ "pos_x": -6704.4375,
+ "pos_y": -156.34375,
+ "pos_z": 14101.875,
+ "stations": "Penal Ship Omicron"
+ },
+ {
+ "name": "Styra",
+ "pos_x": 27.78125,
+ "pos_y": -75.59375,
+ "pos_z": 57.5,
+ "stations": "Bainbridge Installation,Anvil Enterprise"
+ },
+ {
+ "name": "Styx",
+ "pos_x": -24.3125,
+ "pos_y": 37.75,
+ "pos_z": 6.03125,
+ "stations": "Boyle Terminal,Searfoss Plant,Hedley Relay"
+ },
+ {
+ "name": "Su",
+ "pos_x": -27.84375,
+ "pos_y": 88,
+ "pos_z": -63.03125,
+ "stations": "Thagard Port,Melvin Dock,Carrier Colony,Hahn Refinery"
+ },
+ {
+ "name": "Su Li Er",
+ "pos_x": -151.3125,
+ "pos_y": 31.5,
+ "pos_z": -61.09375,
+ "stations": "Baturin's Folly,Wolfe's Pride"
+ },
+ {
+ "name": "Su Lin",
+ "pos_x": 16.40625,
+ "pos_y": -77.3125,
+ "pos_z": 107.625,
+ "stations": "Schoenherr Prospect,Hussenot Station,Card's Progress,Pond Holdings,Scortia Works"
+ },
+ {
+ "name": "Su Lisungu",
+ "pos_x": 141.0625,
+ "pos_y": -109.65625,
+ "pos_z": 97.96875,
+ "stations": "Flamsteed Platform"
+ },
+ {
+ "name": "Subra",
+ "pos_x": 68.21875,
+ "pos_y": 88.0625,
+ "pos_z": -67.5625,
+ "stations": "Jahn Station,Bloomfield Depot"
+ },
+ {
+ "name": "Succelli",
+ "pos_x": 77.59375,
+ "pos_y": -38.375,
+ "pos_z": 47.75,
+ "stations": "McCormick Orbital"
+ },
+ {
+ "name": "Sucha",
+ "pos_x": 66.125,
+ "pos_y": 129.375,
+ "pos_z": -9.625,
+ "stations": "Heck Base,Rothman Base"
+ },
+ {
+ "name": "Suchagga",
+ "pos_x": -56.34375,
+ "pos_y": 23.71875,
+ "pos_z": 56.6875,
+ "stations": "Sherrington Platform"
+ },
+ {
+ "name": "Suchi",
+ "pos_x": -30.25,
+ "pos_y": 18.53125,
+ "pos_z": -121,
+ "stations": "Durrance Port,Cleave Survey,Yourkevitch Settlement"
+ },
+ {
+ "name": "Suchifuku",
+ "pos_x": -125.5625,
+ "pos_y": 8.09375,
+ "pos_z": 52.9375,
+ "stations": "Sverdrup's Progress,Shaikh Mine,Dunyach Landing"
+ },
+ {
+ "name": "Suddene",
+ "pos_x": 83.8125,
+ "pos_y": -152.375,
+ "pos_z": 4.78125,
+ "stations": "Sinclair Exchange,Darren Bowles's Station"
+ },
+ {
+ "name": "Sudi",
+ "pos_x": 23.40625,
+ "pos_y": -51.0625,
+ "pos_z": -85.625,
+ "stations": "Carey Hub,Ross Gateway,Smith Orbital,Conklin Point"
+ },
+ {
+ "name": "Sudihila",
+ "pos_x": 127.46875,
+ "pos_y": -65.71875,
+ "pos_z": 121.15625,
+ "stations": "Lundmark Prospect,Tyson Prospect,Okuni Settlement"
+ },
+ {
+ "name": "Sudii",
+ "pos_x": 139.9375,
+ "pos_y": 14,
+ "pos_z": 19.78125,
+ "stations": "Melvill Vision,Surayev Horizons,Sherrington Station,Dalmas Base,Curbeam Penal colony"
+ },
+ {
+ "name": "Sudini",
+ "pos_x": -80.25,
+ "pos_y": -76.96875,
+ "pos_z": 134.75,
+ "stations": "Henize Port,Forrester Vision"
+ },
+ {
+ "name": "Sudz",
+ "pos_x": -9.875,
+ "pos_y": 52.4375,
+ "pos_z": -29.25,
+ "stations": "Linge Installation,Conway Bastion,Houssay Ring,Ryumin Reach"
+ },
+ {
+ "name": "Suessa",
+ "pos_x": 77.125,
+ "pos_y": -195.4375,
+ "pos_z": -74.3125,
+ "stations": "de Kamp Terminal,Ziegel Landing,Cartmill Penal colony,Shaw Prospect"
+ },
+ {
+ "name": "Suessetani",
+ "pos_x": -44.21875,
+ "pos_y": 37.65625,
+ "pos_z": 104.40625,
+ "stations": "Gutierrez Orbital,Huygens Plant,Whitney Colony"
+ },
+ {
+ "name": "Suessi",
+ "pos_x": 29.1875,
+ "pos_y": 145.125,
+ "pos_z": 24.65625,
+ "stations": "Kandel Platform"
+ },
+ {
+ "name": "Suessina",
+ "pos_x": 74.125,
+ "pos_y": -153.9375,
+ "pos_z": 136.53125,
+ "stations": "Hirn Vision,Barr Depot"
+ },
+ {
+ "name": "Sugag",
+ "pos_x": 84.71875,
+ "pos_y": -59.96875,
+ "pos_z": 138.28125,
+ "stations": "Young Sanctuary,Utkin Installation"
+ },
+ {
+ "name": "Sugagarr",
+ "pos_x": 100.3125,
+ "pos_y": -180.25,
+ "pos_z": 166.28125,
+ "stations": "Barcelona Prospect"
+ },
+ {
+ "name": "Sugal",
+ "pos_x": -38.3125,
+ "pos_y": 68.9375,
+ "pos_z": 117.40625,
+ "stations": "Nye Market,Ali Terminal,Vian Orbital,Hennen Enterprise,Newton Settlement,Forrester Relay,Scott Hub,Simmons Enterprise,al-Haytham Stop,Satcher Port,Harrison Terminal"
+ },
+ {
+ "name": "Sugalis",
+ "pos_x": -158.4375,
+ "pos_y": -41.4375,
+ "pos_z": -23.0625,
+ "stations": "Bachman Port"
+ },
+ {
+ "name": "Sugana",
+ "pos_x": -52.03125,
+ "pos_y": -64.6875,
+ "pos_z": -59.03125,
+ "stations": "Nowak Dock"
+ },
+ {
+ "name": "Sugrivik",
+ "pos_x": 27.59375,
+ "pos_y": -61.125,
+ "pos_z": 19.625,
+ "stations": "Delbruck Dock,Grechko Ring,Goddard Gateway,Maddox Relay,Hernandez Observatory,Archambault Installation"
+ },
+ {
+ "name": "Sugrivitra",
+ "pos_x": -1.96875,
+ "pos_y": 60.3125,
+ "pos_z": 144.3125,
+ "stations": "J. G. Ballard Dock,Alvarez de Pineda Dock,Kneale Landing"
+ },
+ {
+ "name": "Sugrivoruai",
+ "pos_x": 99.84375,
+ "pos_y": -185.25,
+ "pos_z": -13.09375,
+ "stations": "Hipparchus Settlement"
+ },
+ {
+ "name": "Suhte",
+ "pos_x": -168.90625,
+ "pos_y": 27.375,
+ "pos_z": -55.375,
+ "stations": "Ballard Survey,Benyovszky Survey,Fanning Depot,Bester Prospect,Stevens Forum"
+ },
+ {
+ "name": "Sui Guei",
+ "pos_x": -74.78125,
+ "pos_y": -12.84375,
+ "pos_z": 66.375,
+ "stations": "Sleator Relay,Alcala Dock,Brash's Progress,Macquorn Rankine Enterprise,Barratt Port,Pascal Orbital,Curbeam Hub,Soddy Enterprise,Neumann Hub,Fettman Orbital"
+ },
+ {
+ "name": "Sui Redd",
+ "pos_x": -53.1875,
+ "pos_y": 29.5,
+ "pos_z": -120.59375,
+ "stations": "Griggs Station,Solovyev Relay"
+ },
+ {
+ "name": "Sui Ren",
+ "pos_x": 173.96875,
+ "pos_y": -105.84375,
+ "pos_z": -6.53125,
+ "stations": "al-Din al-Urdi Station,Crossfield Mines,Lehtonen's Progress"
+ },
+ {
+ "name": "Sui Xenates",
+ "pos_x": -79.8125,
+ "pos_y": -9.625,
+ "pos_z": 77.75,
+ "stations": "Weyl Platform"
+ },
+ {
+ "name": "Sui Xianoan",
+ "pos_x": -9.15625,
+ "pos_y": -155.4375,
+ "pos_z": -12.09375,
+ "stations": "Purbach Vision,Lassell Ring,Greenleaf Relay"
+ },
+ {
+ "name": "Sui Xiao Gu",
+ "pos_x": 120.5625,
+ "pos_y": -18.28125,
+ "pos_z": 35.34375,
+ "stations": "Heng Dock"
+ },
+ {
+ "name": "Sui Xing",
+ "pos_x": -16.34375,
+ "pos_y": -12.59375,
+ "pos_z": -69.59375,
+ "stations": "Morgan Terminal,Oswald Platform"
+ },
+ {
+ "name": "Suijin",
+ "pos_x": -15.21875,
+ "pos_y": -79.34375,
+ "pos_z": 56.03125,
+ "stations": "Sinclair Vision,Sarafanov Horizons"
+ },
+ {
+ "name": "Sukree",
+ "pos_x": -122.25,
+ "pos_y": 19.71875,
+ "pos_z": 38.46875,
+ "stations": "Dampier Dock,Campbell Observatory,Artin Silo"
+ },
+ {
+ "name": "Sukte",
+ "pos_x": 17.03125,
+ "pos_y": -49.8125,
+ "pos_z": 126.40625,
+ "stations": "Mitchell Orbital,He Enterprise,Reis Enterprise,Narbeth Prospect,Arai Beacon"
+ },
+ {
+ "name": "Suku Maola",
+ "pos_x": -40.0625,
+ "pos_y": 100.25,
+ "pos_z": -100.96875,
+ "stations": "Pangborn Colony,Longyear Plant,Wallace Horizons"
+ },
+ {
+ "name": "Sukua",
+ "pos_x": -42.15625,
+ "pos_y": -51.59375,
+ "pos_z": 171.15625,
+ "stations": "Forest Depot,Wang Base"
+ },
+ {
+ "name": "Sukula",
+ "pos_x": 158.21875,
+ "pos_y": -168.5,
+ "pos_z": -17.96875,
+ "stations": "Heinkel Depot"
+ },
+ {
+ "name": "Sukuluska",
+ "pos_x": 8.125,
+ "pos_y": -25.15625,
+ "pos_z": -151.59375,
+ "stations": "Dantec Terminal,Hannu Enterprise"
+ },
+ {
+ "name": "Sukurbago",
+ "pos_x": 150.9375,
+ "pos_y": -7.90625,
+ "pos_z": -99.4375,
+ "stations": "Faraday City,Sekowski Camp,Volta Terminal,Leclerc Gateway,Neff Penal colony"
+ },
+ {
+ "name": "Sukuruaco",
+ "pos_x": 69.46875,
+ "pos_y": 45.875,
+ "pos_z": 13.6875,
+ "stations": "Nikitin Terminal,Offutt Colony,Charnas Dock,Shiner Vision"
+ },
+ {
+ "name": "Sumarr",
+ "pos_x": 33.40625,
+ "pos_y": 30.21875,
+ "pos_z": -40.21875,
+ "stations": "Armstrong Station"
+ },
+ {
+ "name": "Sumel",
+ "pos_x": -74.8125,
+ "pos_y": -5.65625,
+ "pos_z": 69.84375,
+ "stations": "Kingsmill Refinery,Christian Prospect"
+ },
+ {
+ "name": "Sumeragwa",
+ "pos_x": 197.9375,
+ "pos_y": -146.59375,
+ "pos_z": 70.46875,
+ "stations": "Dean Depot,Elmore Plant,Pickering Dock"
+ },
+ {
+ "name": "Sumeru",
+ "pos_x": -136.1875,
+ "pos_y": -40.5,
+ "pos_z": 28.875,
+ "stations": "Pasteur Settlement"
+ },
+ {
+ "name": "Sumeya",
+ "pos_x": 62.65625,
+ "pos_y": 40.34375,
+ "pos_z": -42.875,
+ "stations": "Weitz Mine,Burnell Plant,Lawson Plant"
+ },
+ {
+ "name": "Sumia",
+ "pos_x": 24.5625,
+ "pos_y": -100.5,
+ "pos_z": 165.78125,
+ "stations": "Mikoyan Orbital,Ryan Survey,Ikeya Settlement,Pinto Landing"
+ },
+ {
+ "name": "Summanus",
+ "pos_x": 52.1875,
+ "pos_y": -34.625,
+ "pos_z": -0.6875,
+ "stations": "Carlisle Estate,Caidin Hub,Lobachevsky Dock,Payson Depot,Wankel Holdings"
+ },
+ {
+ "name": "Summerland",
+ "pos_x": 28.9375,
+ "pos_y": -121.09375,
+ "pos_z": 3.53125,
+ "stations": "Henry O'Hare's Hangar,Somerville Terminal,van Rhijn Hub,Muller Terminal,Shoemaker Point,Henry O'Hare's Hanger"
+ },
+ {
+ "name": "Sumo",
+ "pos_x": -51.34375,
+ "pos_y": 143,
+ "pos_z": 82,
+ "stations": "Korzun Outpost,Glidden Outpost"
+ },
+ {
+ "name": "Sumod",
+ "pos_x": -60.375,
+ "pos_y": -77.8125,
+ "pos_z": 45.625,
+ "stations": "Dashiell Dock,Mallett Orbital,Sharp Dock,Brunel Mine,Popovich Exchange,Lee Enterprise"
+ },
+ {
+ "name": "Sumokuth",
+ "pos_x": -37.0625,
+ "pos_y": -128.4375,
+ "pos_z": 97.09375,
+ "stations": "Pei Settlement,Foster Vision"
+ },
+ {
+ "name": "Sun Aphu",
+ "pos_x": -166.1875,
+ "pos_y": -3.78125,
+ "pos_z": -33.46875,
+ "stations": "Lavrador Survey"
+ },
+ {
+ "name": "Sun Batha",
+ "pos_x": 49.6875,
+ "pos_y": -92.75,
+ "pos_z": 170.65625,
+ "stations": "Naboth Beacon"
+ },
+ {
+ "name": "Sun Bin",
+ "pos_x": -118.1875,
+ "pos_y": -18.21875,
+ "pos_z": 7.4375,
+ "stations": "Mills Settlement,Melnick Base,Gurney Survey"
+ },
+ {
+ "name": "Sun Chem",
+ "pos_x": -67.3125,
+ "pos_y": 136.09375,
+ "pos_z": 13.59375,
+ "stations": "Varthema Relay"
+ },
+ {
+ "name": "Sun Chuen",
+ "pos_x": 124.03125,
+ "pos_y": -74.375,
+ "pos_z": -0.34375,
+ "stations": "Bolger's Pride,Bryant Orbital"
+ },
+ {
+ "name": "Sun Pef",
+ "pos_x": 161.90625,
+ "pos_y": -10.84375,
+ "pos_z": 13.8125,
+ "stations": "Tousey Port,Skjellerup Vision"
+ },
+ {
+ "name": "Sun Pi",
+ "pos_x": 82.71875,
+ "pos_y": 38.5,
+ "pos_z": 106.4375,
+ "stations": "Lethem Orbital,Russ Enterprise,Okorafor Vision,Linaweaver Enterprise,Gann Penal colony"
+ },
+ {
+ "name": "Sun Picts",
+ "pos_x": 61.28125,
+ "pos_y": -119.1875,
+ "pos_z": -71.25,
+ "stations": "Honda Works,Rubin Prospect"
+ },
+ {
+ "name": "Sun Pin",
+ "pos_x": 68.1875,
+ "pos_y": 57.15625,
+ "pos_z": -43.3125,
+ "stations": "Freud Installation,Elder Silo"
+ },
+ {
+ "name": "Sun Takush",
+ "pos_x": -54.53125,
+ "pos_y": -17.21875,
+ "pos_z": 57.59375,
+ "stations": "McNair Gateway"
+ },
+ {
+ "name": "Sun Tsanapi",
+ "pos_x": 95.46875,
+ "pos_y": 21.46875,
+ "pos_z": -102.5,
+ "stations": "Dedekind Legacy"
+ },
+ {
+ "name": "Sun Wen",
+ "pos_x": -36.90625,
+ "pos_y": 51.09375,
+ "pos_z": -67.5625,
+ "stations": "Freud Gateway,Clair Survey,Nasmyth Terminal,Soddy Terminal,Person Base"
+ },
+ {
+ "name": "Sun Wen Ho",
+ "pos_x": -201.1875,
+ "pos_y": -30.8125,
+ "pos_z": 68.15625,
+ "stations": "Rosenberg Point,Ings Camp"
+ },
+ {
+ "name": "Sungai",
+ "pos_x": 40.65625,
+ "pos_y": -24.34375,
+ "pos_z": 58.8125,
+ "stations": "Willis Station,Treshchov Platform,Forest Park,Alas Settlement,Readdy Park"
+ },
+ {
+ "name": "Supay",
+ "pos_x": -91.46875,
+ "pos_y": 57.5,
+ "pos_z": -0.3125,
+ "stations": "Laveykin Hub,Crown Orbital,Yurchikhin City,Wood Depot,Smith Bastion"
+ },
+ {
+ "name": "Supen",
+ "pos_x": -95.25,
+ "pos_y": 61.53125,
+ "pos_z": -47.71875,
+ "stations": "Sevastyanov Station,Ellern Arsenal"
+ },
+ {
+ "name": "Supenges",
+ "pos_x": 100.625,
+ "pos_y": -215.28125,
+ "pos_z": -16.15625,
+ "stations": "Mobius Stop"
+ },
+ {
+ "name": "Supera",
+ "pos_x": -131.375,
+ "pos_y": -49.53125,
+ "pos_z": 42.3125,
+ "stations": "Garfinkle Platform,Evans Landing"
+ },
+ {
+ "name": "Superty",
+ "pos_x": 35.59375,
+ "pos_y": -12.6875,
+ "pos_z": 80.90625,
+ "stations": "Hendel Point,Hamilton Landing"
+ },
+ {
+ "name": "Sura Rama",
+ "pos_x": 93.375,
+ "pos_y": -93.21875,
+ "pos_z": 101.53125,
+ "stations": "Anderson Terminal,Kuiper Bastion"
+ },
+ {
+ "name": "Suraci",
+ "pos_x": -102.53125,
+ "pos_y": 9.34375,
+ "pos_z": -51.625,
+ "stations": "Edwards Port,Boming Dock,Wolfe Survey,Siegel Observatory,O'Brien Point"
+ },
+ {
+ "name": "Surmati",
+ "pos_x": 24.09375,
+ "pos_y": 1.5,
+ "pos_z": 76.5625,
+ "stations": "Mendeleev Works"
+ },
+ {
+ "name": "Surmodi",
+ "pos_x": -42.15625,
+ "pos_y": 82.03125,
+ "pos_z": 129.96875,
+ "stations": "Tuan Port"
+ },
+ {
+ "name": "Surti",
+ "pos_x": 67.71875,
+ "pos_y": -23.09375,
+ "pos_z": -94.0625,
+ "stations": "Vercors Hub,Roberts Keep,Leinster Holdings"
+ },
+ {
+ "name": "Surtiong",
+ "pos_x": 164.46875,
+ "pos_y": -42.84375,
+ "pos_z": -50.34375,
+ "stations": "Norman Arena,Phillips' Claim,Brosnan Vision"
+ },
+ {
+ "name": "Surya",
+ "pos_x": -38.46875,
+ "pos_y": 39.25,
+ "pos_z": 5.40625,
+ "stations": "Burbank Camp,Hirase Terminal,Arnarson Prospect"
+ },
+ {
+ "name": "Susa",
+ "pos_x": -22.9375,
+ "pos_y": 72.125,
+ "pos_z": -113.84375,
+ "stations": "Crook Ring"
+ },
+ {
+ "name": "Susala",
+ "pos_x": -124.40625,
+ "pos_y": 41.4375,
+ "pos_z": 87.25,
+ "stations": "Popper Station,Teng Port,Keyes Enterprise,Scott Hub"
+ },
+ {
+ "name": "Susama",
+ "pos_x": -134.5,
+ "pos_y": 35.28125,
+ "pos_z": -26.21875,
+ "stations": "Mullane Beacon,Kaku Dock,Tudela's Claim"
+ },
+ {
+ "name": "Susan",
+ "pos_x": -7.0625,
+ "pos_y": 59,
+ "pos_z": 107.84375,
+ "stations": "Forsskal Mine,Malerba Penal colony"
+ },
+ {
+ "name": "Susang",
+ "pos_x": -162.4375,
+ "pos_y": -13.15625,
+ "pos_z": 57.15625,
+ "stations": "Harrison Platform"
+ },
+ {
+ "name": "Susano",
+ "pos_x": 133.1875,
+ "pos_y": -209.71875,
+ "pos_z": -19.34375,
+ "stations": "Jackson Gateway,Laumer Base,Sternbach Terminal"
+ },
+ {
+ "name": "Susanoo",
+ "pos_x": 12.1875,
+ "pos_y": -1.65625,
+ "pos_z": -62.78125,
+ "stations": "Huygens Mines"
+ },
+ {
+ "name": "Suss",
+ "pos_x": -135.25,
+ "pos_y": 34.0625,
+ "pos_z": 56.78125,
+ "stations": "Szilard Orbital,Jensen Prospect,McCoy Installation,Longyear Lab"
+ },
+ {
+ "name": "Sussche",
+ "pos_x": -45.78125,
+ "pos_y": -17,
+ "pos_z": -90.28125,
+ "stations": "Lefschetz Holdings,Laliberte Depot"
+ },
+ {
+ "name": "Suta",
+ "pos_x": 92.9375,
+ "pos_y": 42.03125,
+ "pos_z": -18.40625,
+ "stations": "Mitchell Enterprise,Mitchell Landing,Moon Relay,Adamson Base"
+ },
+ {
+ "name": "Sutei",
+ "pos_x": 55.40625,
+ "pos_y": 108.84375,
+ "pos_z": -5.5,
+ "stations": "Riemann Beacon,McQuay Prospect,Kratman Dock,Krikalev Mine"
+ },
+ {
+ "name": "Sutekh",
+ "pos_x": -29.53125,
+ "pos_y": -50.78125,
+ "pos_z": 3.125,
+ "stations": "Archimedes Gateway,Williamson Keep,Rorschach City,Cormack Vision"
+ },
+ {
+ "name": "Suteni",
+ "pos_x": 107.09375,
+ "pos_y": -135.71875,
+ "pos_z": -30.65625,
+ "stations": "Dassault Orbital,Foss Vision,Merrill Hub,Burroughs Reach,Pacheco Installation,Smith Beacon"
+ },
+ {
+ "name": "Suteruku",
+ "pos_x": 9.96875,
+ "pos_y": -147.90625,
+ "pos_z": 61.28125,
+ "stations": "Charlois Station,Ahmed Survey,Jenkins Base,Baillaud Station,Maunder Hub"
+ },
+ {
+ "name": "Suttiren",
+ "pos_x": 98.78125,
+ "pos_y": -214.0625,
+ "pos_z": 65.03125,
+ "stations": "Pu Orbital,Matthaus Olbers Platform,Shepherd Prospect"
+ },
+ {
+ "name": "Suttonenses",
+ "pos_x": -102.96875,
+ "pos_y": 57.375,
+ "pos_z": -21.9375,
+ "stations": "Fujimori Survey,Lindsey Dock,Hutchinson Horizons,Kopra's Folly"
+ },
+ {
+ "name": "Suttora",
+ "pos_x": 113,
+ "pos_y": -9.03125,
+ "pos_z": -81,
+ "stations": "Wittgenstein Station,Good Settlement"
+ },
+ {
+ "name": "Suttundjina",
+ "pos_x": 107.46875,
+ "pos_y": -144.375,
+ "pos_z": -11.84375,
+ "stations": "Kidinnu Landing,Mies van der Rohe Mines,Oltion Point"
+ },
+ {
+ "name": "Suya",
+ "pos_x": -122.125,
+ "pos_y": -51.09375,
+ "pos_z": 48.6875,
+ "stations": "Rutherford Port,Houtman Survey,Scheerbart Beacon"
+ },
+ {
+ "name": "Suyar",
+ "pos_x": -51.90625,
+ "pos_y": -112.71875,
+ "pos_z": 87.9375,
+ "stations": "Galton Colony,Flade Dock"
+ },
+ {
+ "name": "Suyarang",
+ "pos_x": 21.15625,
+ "pos_y": -12.0625,
+ "pos_z": -75.84375,
+ "stations": "Volta Ring,Treshchov Orbital,Ordway's Inheritance,Shaw Terminal"
+ },
+ {
+ "name": "Svalinn",
+ "pos_x": -96.4375,
+ "pos_y": 10.4375,
+ "pos_z": 38.1875,
+ "stations": "Khayyam Dock,Czerneda Base"
+ },
+ {
+ "name": "Svandi",
+ "pos_x": -141.59375,
+ "pos_y": 79.875,
+ "pos_z": 5.0625,
+ "stations": "Frechet Base,Margulies Arena"
+ },
+ {
+ "name": "Svandovit",
+ "pos_x": -25.21875,
+ "pos_y": 115.28125,
+ "pos_z": -97.78125,
+ "stations": "Filipchenko Terminal"
+ },
+ {
+ "name": "Svanequixil",
+ "pos_x": 28.34375,
+ "pos_y": -197.84375,
+ "pos_z": 132.71875,
+ "stations": "Peltier Landing"
+ },
+ {
+ "name": "Svansa",
+ "pos_x": -147.78125,
+ "pos_y": -66.34375,
+ "pos_z": -98.34375,
+ "stations": "Xuanzang Enterprise,Laing Mines,Przhevalsky Hub"
+ },
+ {
+ "name": "Svantetit",
+ "pos_x": -141.1875,
+ "pos_y": 44.5,
+ "pos_z": 7.59375,
+ "stations": "Kurland Prospect,O'Leary Outpost,Low Beacon"
+ },
+ {
+ "name": "Svanticani",
+ "pos_x": -24.46875,
+ "pos_y": -63.875,
+ "pos_z": 46.15625,
+ "stations": "Pierres Mine,King Port,Carpini Survey,Sargent Terminal,Campbell Refinery"
+ },
+ {
+ "name": "Svantiochna",
+ "pos_x": -102,
+ "pos_y": 75.5625,
+ "pos_z": -57.21875,
+ "stations": "Collins Depot,Nehsi Relay"
+ },
+ {
+ "name": "Svara",
+ "pos_x": 56.40625,
+ "pos_y": -35.125,
+ "pos_z": -67.53125,
+ "stations": "Griffin Dock,Onufrienko Barracks,Barnes City,Samuda Park,Walters Installation"
+ },
+ {
+ "name": "Svaradareco",
+ "pos_x": -58.375,
+ "pos_y": -62.34375,
+ "pos_z": -151.96875,
+ "stations": "Quimper Works"
+ },
+ {
+ "name": "Svaratia",
+ "pos_x": -14.53125,
+ "pos_y": -71.96875,
+ "pos_z": -80.40625,
+ "stations": "Bouch Orbital,Gernsback Keep,Chu Landing,Gaultier de Varennes Base"
+ },
+ {
+ "name": "Svari",
+ "pos_x": 92.40625,
+ "pos_y": -151.46875,
+ "pos_z": 28.71875,
+ "stations": "Michalitsianos Dock,Lane Vision,Narlikar Orbital"
+ },
+ {
+ "name": "Svarindbh",
+ "pos_x": -61.21875,
+ "pos_y": 36.8125,
+ "pos_z": 163.03125,
+ "stations": "White Beacon"
+ },
+ {
+ "name": "Svarizic",
+ "pos_x": 28.125,
+ "pos_y": 110.0625,
+ "pos_z": 36.34375,
+ "stations": "Galouye Orbital"
+ },
+ {
+ "name": "Svarogich",
+ "pos_x": 114.34375,
+ "pos_y": -203.78125,
+ "pos_z": 101.53125,
+ "stations": "Honda Terminal,Kurtz Platform,Boss Keep"
+ },
+ {
+ "name": "Svaroo",
+ "pos_x": 105.21875,
+ "pos_y": -100.09375,
+ "pos_z": -51.875,
+ "stations": "Ahnert Station"
+ },
+ {
+ "name": "Svarth",
+ "pos_x": 38.28125,
+ "pos_y": 116.78125,
+ "pos_z": 38.65625,
+ "stations": "Shkaplerov Colony"
+ },
+ {
+ "name": "Svass",
+ "pos_x": 29.9375,
+ "pos_y": -82.59375,
+ "pos_z": -40.53125,
+ "stations": "Reilly's Retreat,Bereznyak City,Maria's Rest,Aikin Enterprise"
+ },
+ {
+ "name": "Sveinbarn",
+ "pos_x": -35.6875,
+ "pos_y": 21.375,
+ "pos_z": 89.21875,
+ "stations": "Cugnot Orbital,Bunch Point,Wallace Hub,Johnson Enterprise,Godwin Prospect"
+ },
+ {
+ "name": "Sveit",
+ "pos_x": 51,
+ "pos_y": 20.3125,
+ "pos_z": 82.6875,
+ "stations": "Barentsz Orbital,Heceta Dock,Green Base,Akers Installation,Santarem Stop,Currie Prospect,Malcolm's Folly"
+ },
+ {
+ "name": "Svent",
+ "pos_x": -12.71875,
+ "pos_y": 19.84375,
+ "pos_z": 103.15625,
+ "stations": "Galindo Hub,Capek Gateway,Bunnell Beacon,Bamford Base"
+ },
+ {
+ "name": "Svitra",
+ "pos_x": -55.34375,
+ "pos_y": 9.25,
+ "pos_z": 153.75,
+ "stations": "Fung Landing,Pryor Arsenal"
+ },
+ {
+ "name": "Swahili",
+ "pos_x": 168.4375,
+ "pos_y": -77.75,
+ "pos_z": 121,
+ "stations": "Coggia Hub,Kreutz Enterprise,Maanen Dock"
+ },
+ {
+ "name": "Swahku",
+ "pos_x": 91.25,
+ "pos_y": -74.90625,
+ "pos_z": -126.5625,
+ "stations": "Corte-Real Point,Reilly Legacy,Bunsen Works"
+ },
+ {
+ "name": "Swahukcha",
+ "pos_x": -93.125,
+ "pos_y": -63.5,
+ "pos_z": -20.75,
+ "stations": "Flinders Bastion,Peirce Plant"
+ },
+ {
+ "name": "Swaza",
+ "pos_x": -21.15625,
+ "pos_y": -5.40625,
+ "pos_z": 57.34375,
+ "stations": "Onnes Settlement,Barratt Dock"
+ },
+ {
+ "name": "Swazahua",
+ "pos_x": -91.53125,
+ "pos_y": 102.375,
+ "pos_z": -40.09375,
+ "stations": "Narvaez Terminal,Saberhagen Relay,Dietz Observatory,Dedman Ring"
+ },
+ {
+ "name": "Swazakan",
+ "pos_x": 97.21875,
+ "pos_y": -25.03125,
+ "pos_z": 102.875,
+ "stations": "Acton Silo,Haisheng Hangar"
+ },
+ {
+ "name": "Swazakano",
+ "pos_x": 143.5625,
+ "pos_y": -136.3125,
+ "pos_z": 4.03125,
+ "stations": "Vries' Progress,Fehrenbach Hub,Rich Camp"
+ },
+ {
+ "name": "Syntec",
+ "pos_x": 63.5625,
+ "pos_y": 0.21875,
+ "pos_z": 46.28125,
+ "stations": "Leavitt City,Oersted Gateway,Cartan Settlement,Lawson Ring"
+ },
+ {
+ "name": "Synteini",
+ "pos_x": 51.78125,
+ "pos_y": -76.40625,
+ "pos_z": 28.71875,
+ "stations": "Lagerkvist Gateway,William Sargent Vision"
+ },
+ {
+ "name": "Synten",
+ "pos_x": 26.34375,
+ "pos_y": -24.96875,
+ "pos_z": -143.09375,
+ "stations": "Bruce Stop,Oramus Base"
+ },
+ {
+ "name": "Synteri",
+ "pos_x": -121,
+ "pos_y": -87.53125,
+ "pos_z": 65.65625,
+ "stations": "Fontenay Platform"
+ },
+ {
+ "name": "Syntes",
+ "pos_x": 130.0625,
+ "pos_y": 108.28125,
+ "pos_z": 99.375,
+ "stations": "Swift Orbital,Pytheas Prospect,Brunel Orbital,Denton Survey"
+ },
+ {
+ "name": "Syntha",
+ "pos_x": 85.03125,
+ "pos_y": -11.40625,
+ "pos_z": -132.71875,
+ "stations": "Luiken Mines"
+ },
+ {
+ "name": "Syntheng",
+ "pos_x": 41.75,
+ "pos_y": -156.875,
+ "pos_z": 126.8125,
+ "stations": "Foster Station,Sutcliffe Holdings,Frazetta Colony,Celsius Dock"
+ },
+ {
+ "name": "Synuefai EB-R c7-5",
+ "pos_x": -291.59375,
+ "pos_y": -224.375,
+ "pos_z": -731.09375,
+ "stations": "California Gateway"
+ },
+ {
+ "name": "Synuefai HY-K c24-2",
+ "pos_x": -90.15625,
+ "pos_y": -268.21875,
+ "pos_z": -0.53125,
+ "stations": "Sabine Base"
+ },
+ {
+ "name": "Synuefai LC-C b46-1",
+ "pos_x": -241.25,
+ "pos_y": -202.5,
+ "pos_z": -84.8125,
+ "stations": "Thornycroft Terminal"
+ },
+ {
+ "name": "Synuefai LX-R d5-28",
+ "pos_x": -244.5625,
+ "pos_y": -218.5,
+ "pos_z": -574.96875,
+ "stations": "New Beaumont Dock,Perseus Hold"
+ },
+ {
+ "name": "Synuefai RJ-F d12-51",
+ "pos_x": -89.4375,
+ "pos_y": -286.03125,
+ "pos_z": 13,
+ "stations": "Baker Point"
+ },
+ {
+ "name": "Synuefe BT-F d12-106",
+ "pos_x": 211.5625,
+ "pos_y": -183.84375,
+ "pos_z": -8.59375,
+ "stations": "Quaglia Beacon"
+ },
+ {
+ "name": "Synuefe BT-F d12-54",
+ "pos_x": 228.875,
+ "pos_y": -117.125,
+ "pos_z": 1.6875,
+ "stations": "Daniel's Folly"
+ },
+ {
+ "name": "Synuefe BT-F d12-97",
+ "pos_x": 221.375,
+ "pos_y": -115.1875,
+ "pos_z": 28.625,
+ "stations": "Scheerbart Landing"
+ },
+ {
+ "name": "Synuefe CM-L c24-14",
+ "pos_x": 212.53125,
+ "pos_y": -147.5,
+ "pos_z": -14.96875,
+ "stations": "Gelfand Settlement,Cellarius Enterprise"
+ },
+ {
+ "name": "Synuefe FZ-D d13-122",
+ "pos_x": 214.25,
+ "pos_y": -141.625,
+ "pos_z": 60.625,
+ "stations": "Hunt Terminal"
+ },
+ {
+ "name": "Synuefe FZ-D d13-91",
+ "pos_x": 221.03125,
+ "pos_y": -144.6875,
+ "pos_z": 68.3125,
+ "stations": "Meyrink Base"
+ },
+ {
+ "name": "Synuefe GU-D d13-78",
+ "pos_x": 160.78125,
+ "pos_y": -257.5625,
+ "pos_z": 85.6875,
+ "stations": "Bokeili Survey,Dumont Vision"
+ },
+ {
+ "name": "Synuefe KY-H c26-4",
+ "pos_x": 208.5,
+ "pos_y": -182.5,
+ "pos_z": 86.28125,
+ "stations": "Struzan Landing"
+ },
+ {
+ "name": "Synuefe KY-H c26-5",
+ "pos_x": 208.03125,
+ "pos_y": -179.71875,
+ "pos_z": 88.78125,
+ "stations": "Havilland Terminal"
+ },
+ {
+ "name": "Synuefe RT-R c20-7",
+ "pos_x": 72.28125,
+ "pos_y": -326.71875,
+ "pos_z": -155.125,
+ "stations": "Diamond Vision,Dolgov Point"
+ },
+ {
+ "name": "Synuefe YH-H d11-66",
+ "pos_x": 134.4375,
+ "pos_y": -257.3125,
+ "pos_z": -52.1875,
+ "stations": "Bernoulli Camp"
+ },
+ {
+ "name": "Synuefe YK-N c23-18",
+ "pos_x": 272.15625,
+ "pos_y": -128.03125,
+ "pos_z": -36.75,
+ "stations": "Polya Horizons"
+ },
+ {
+ "name": "Synuefe YK-N c23-8",
+ "pos_x": 277.46875,
+ "pos_y": -123.625,
+ "pos_z": -41.6875,
+ "stations": "Clerk Vision"
+ },
+ {
+ "name": "Synuefe YM-H d11-84",
+ "pos_x": 277.3125,
+ "pos_y": -125.0625,
+ "pos_z": -30.90625,
+ "stations": "Neville Base"
+ },
+ {
+ "name": "Syreadiae JX-F c0",
+ "pos_x": -9529.4375,
+ "pos_y": -64.5,
+ "pos_z": -7428.4375,
+ "stations": "The Zurara"
+ },
+ {
+ "name": "Syrma",
+ "pos_x": 16.9375,
+ "pos_y": 55.96875,
+ "pos_z": 42.875,
+ "stations": "Bartlett Vision,Kagan Platform"
+ },
+ {
+ "name": "T Tauri",
+ "pos_x": -32.96875,
+ "pos_y": -206.40625,
+ "pos_z": -557.3125,
+ "stations": "Hind Mine"
+ },
+ {
+ "name": "T Ti Wari",
+ "pos_x": -2.5625,
+ "pos_y": -133.75,
+ "pos_z": -93.9375,
+ "stations": "Besonders Terminal"
+ },
+ {
+ "name": "T Tie",
+ "pos_x": -11.28125,
+ "pos_y": -154.21875,
+ "pos_z": 36.125,
+ "stations": "Jael Terminal,Budrys Depot,Messerschmitt Orbital,Sukhoi Hangar"
+ },
+ {
+ "name": "T'a Shakarwa",
+ "pos_x": -87.875,
+ "pos_y": -67.53125,
+ "pos_z": -80.59375,
+ "stations": "Low Depot"
+ },
+ {
+ "name": "T'an",
+ "pos_x": -15.875,
+ "pos_y": -121.9375,
+ "pos_z": 16.65625,
+ "stations": "Pennington Terminal,Moskowitz Barracks"
+ },
+ {
+ "name": "T'ao T'an",
+ "pos_x": 76.5,
+ "pos_y": -144.0625,
+ "pos_z": 155.875,
+ "stations": "Flammarion Port,Lindblad Station"
+ },
+ {
+ "name": "T'ao Tzu",
+ "pos_x": 55.5625,
+ "pos_y": -242.9375,
+ "pos_z": -2.5625,
+ "stations": "Campbell Outpost"
+ },
+ {
+ "name": "T'ao Warla",
+ "pos_x": 57.09375,
+ "pos_y": 99.25,
+ "pos_z": -84.9375,
+ "stations": "Savinykh City,Casper Point"
+ },
+ {
+ "name": "T'ien",
+ "pos_x": 136.75,
+ "pos_y": -178.46875,
+ "pos_z": 113.28125,
+ "stations": "Charlois Enterprise,Faye Vision,Urkovic Landing,Danjon City,Sei Penal colony"
+ },
+ {
+ "name": "T'ien Mu",
+ "pos_x": 4.09375,
+ "pos_y": -67.65625,
+ "pos_z": -73.1875,
+ "stations": "Deere Platform,Grabe Ring,Sacco Gateway"
+ },
+ {
+ "name": "T'ieni",
+ "pos_x": 50.46875,
+ "pos_y": 96.75,
+ "pos_z": 87.96875,
+ "stations": "Vetulani Port,von Krusenstern Bastion,Phillifent's Progress"
+ },
+ {
+ "name": "T'ienimi",
+ "pos_x": -120.71875,
+ "pos_y": 18,
+ "pos_z": -116.0625,
+ "stations": "Barnaby Terminal,Wolf Landing,Fontana Port,Turzillo Depot"
+ },
+ {
+ "name": "T'iensei",
+ "pos_x": 18.34375,
+ "pos_y": -50.75,
+ "pos_z": 113.28125,
+ "stations": "Schmitt Port,Dzhanibekov Port,Whymper Vision,Xing Terminal,Love Ring,He Stop,Ray Hub,Jemison Terminal,Ejeta City,Cori Port,Hennen Dock,McIntyre's Claim,Jacobi Oasis,Farmer Depot,Tshang's Folly,Everest Horizons"
+ },
+ {
+ "name": "T'u Koji",
+ "pos_x": -4.4375,
+ "pos_y": -113.5625,
+ "pos_z": 115.03125,
+ "stations": "Paez Lab,Mrkos Relay,Walotsky Orbital,Howe Mines,Baxter Works"
+ },
+ {
+ "name": "T'u Tie",
+ "pos_x": -127.125,
+ "pos_y": -88.125,
+ "pos_z": 77.5625,
+ "stations": "Rubruck Colony,Euthymenes Lab"
+ },
+ {
+ "name": "T'u Tu",
+ "pos_x": 107.6875,
+ "pos_y": -101.03125,
+ "pos_z": 59.75,
+ "stations": "Akiyama City,Parsons Orbital,Evans Orbital,Homer Port,Anderson Prospect,Tem's Inheritance,Varley Reach,Quetelet City,Fallows Hub,Dalgarno Port,Palitzsch Port,Mies van der Rohe Orbital"
+ },
+ {
+ "name": "Tabajara",
+ "pos_x": 105.34375,
+ "pos_y": 74.40625,
+ "pos_z": 120.03125,
+ "stations": "Merchiston's Folly,Anderson Point"
+ },
+ {
+ "name": "Tabala",
+ "pos_x": -82.46875,
+ "pos_y": -60.46875,
+ "pos_z": 61,
+ "stations": "Stafford Orbital,Watts Silo,Ellern Enterprise,Wisoff Stop"
+ },
+ {
+ "name": "Tabalban",
+ "pos_x": 114.125,
+ "pos_y": -48.3125,
+ "pos_z": -13.375,
+ "stations": "Cochrane Plant,Howard Horizons"
+ },
+ {
+ "name": "Tabaldak",
+ "pos_x": 26,
+ "pos_y": -121.84375,
+ "pos_z": 87.90625,
+ "stations": "Drake Orbital,Rogers Dock,Danforth City,Cowling Ring,Shen Station,Clauss' Progress"
+ },
+ {
+ "name": "Tabassapisi",
+ "pos_x": -84.5,
+ "pos_y": 37.34375,
+ "pos_z": -2.25,
+ "stations": "Dobzhansky Port,Clair Gateway,Parker Enterprise,Stott Gateway,Hovgaard Prospect"
+ },
+ {
+ "name": "Tabaye",
+ "pos_x": 68.5625,
+ "pos_y": -212.625,
+ "pos_z": -3.5625,
+ "stations": "Uto Orbital"
+ },
+ {
+ "name": "Tabit",
+ "pos_x": 4.9375,
+ "pos_y": -10.0625,
+ "pos_z": -23.8125,
+ "stations": "Haber City,Garfinkle Hub,Bridger Installation,Pinto Hub"
+ },
+ {
+ "name": "Tabwa",
+ "pos_x": 80.1875,
+ "pos_y": -7.65625,
+ "pos_z": 142.15625,
+ "stations": "Wallis Enterprise,Fairbairn City,Goldstein Port,Hoffman Relay,Park Landing"
+ },
+ {
+ "name": "Tacabs",
+ "pos_x": 20.21875,
+ "pos_y": -261.90625,
+ "pos_z": 9.96875,
+ "stations": "Hirayama Dock,Kranz Station,Meredith Vision,Sitterly Mines"
+ },
+ {
+ "name": "Tacae",
+ "pos_x": -50.1875,
+ "pos_y": 82.84375,
+ "pos_z": -14.15625,
+ "stations": "Due Prospect"
+ },
+ {
+ "name": "Tacahuti",
+ "pos_x": 160.1875,
+ "pos_y": -128.59375,
+ "pos_z": 41.5,
+ "stations": "Jones Terminal,Perlmutter Station,Houten-Groeneveld Hub,Gabrielli Settlement"
+ },
+ {
+ "name": "Tacajandi",
+ "pos_x": 54.40625,
+ "pos_y": -124.46875,
+ "pos_z": -98.125,
+ "stations": "Bailey Holdings,Rosenberg Hub,Tsunenaga Port,Stefansson Installation"
+ },
+ {
+ "name": "Tacana",
+ "pos_x": -87.71875,
+ "pos_y": 102.71875,
+ "pos_z": -1.65625,
+ "stations": "Gwynn Plant,Knight Vision"
+ },
+ {
+ "name": "Tacare",
+ "pos_x": 46.09375,
+ "pos_y": -73.84375,
+ "pos_z": -149.625,
+ "stations": "Clifford Terminal"
+ },
+ {
+ "name": "Tachmetae",
+ "pos_x": -0.59375,
+ "pos_y": 60.6875,
+ "pos_z": 84.71875,
+ "stations": "Mills Beacon,Bresnik Station,Brandenstein Ring,Bosch Enterprise"
+ },
+ {
+ "name": "Taevaisa",
+ "pos_x": 77.9375,
+ "pos_y": -133.90625,
+ "pos_z": 16,
+ "stations": "Sopwith Arsenal,Derekas Installation,Frankal"
+ },
+ {
+ "name": "Taexali",
+ "pos_x": 33.5,
+ "pos_y": -164.71875,
+ "pos_z": 127.9375,
+ "stations": "Kotelnikov Enterprise,Alden Ring,Baynes Survey,Mattingly Hub"
+ },
+ {
+ "name": "Taexalis",
+ "pos_x": -29.84375,
+ "pos_y": -171.25,
+ "pos_z": 60.5625,
+ "stations": "Lassell Settlement"
+ },
+ {
+ "name": "TaexaliTemu",
+ "pos_x": 47.6875,
+ "pos_y": -154.28125,
+ "pos_z": 106.375,
+ "stations": "Xuesen Dock,Bayer Terminal,Bonestell Orbital"
+ },
+ {
+ "name": "Taexalk",
+ "pos_x": 109.28125,
+ "pos_y": -11.6875,
+ "pos_z": -73.75,
+ "stations": "Kurland Station,Euthymenes Station,Moskowitz Depot,Reiter Vision,Riemann Bastion"
+ },
+ {
+ "name": "Taexalka",
+ "pos_x": 59.625,
+ "pos_y": -1.34375,
+ "pos_z": 160.90625,
+ "stations": "Beliaev Terminal,Caidin Hub,Shapiro Bastion"
+ },
+ {
+ "name": "Taga",
+ "pos_x": -20.625,
+ "pos_y": 14.625,
+ "pos_z": 142.875,
+ "stations": "Jun Station,Gamow Colony,Moorcock's Claim"
+ },
+ {
+ "name": "Tagalag",
+ "pos_x": 96.65625,
+ "pos_y": -201.75,
+ "pos_z": 79.34375,
+ "stations": "Seki Point"
+ },
+ {
+ "name": "Tagarilocan",
+ "pos_x": 117,
+ "pos_y": -112.6875,
+ "pos_z": 153.1875,
+ "stations": "Nijland Camp"
+ },
+ {
+ "name": "Tagii",
+ "pos_x": 8.40625,
+ "pos_y": -59.71875,
+ "pos_z": 164.25,
+ "stations": "Schweinfurth Legacy,Vinge Dock,Galindo Survey"
+ },
+ {
+ "name": "Tagin",
+ "pos_x": -140.21875,
+ "pos_y": -48.15625,
+ "pos_z": -44.875,
+ "stations": "Peirce Point"
+ },
+ {
+ "name": "Tago",
+ "pos_x": -1.53125,
+ "pos_y": 68.15625,
+ "pos_z": 74.21875,
+ "stations": "Howe Terminal,Hackworth City,Makarov Terminal,MacLean Ring,Brash Ring,Baker Enterprise,Molina Gateway,Kregel Dock,Hale Installation"
+ },
+ {
+ "name": "Tagoman",
+ "pos_x": -3.1875,
+ "pos_y": -160.1875,
+ "pos_z": 19.5625,
+ "stations": "Brorsen Port,Laurent Ring,Quetelet Gateway,Varley Hub"
+ },
+ {
+ "name": "Tagomane",
+ "pos_x": 61.4375,
+ "pos_y": 29.5625,
+ "pos_z": -141.5625,
+ "stations": "Thomas Dock,Narita Vista"
+ },
+ {
+ "name": "Taha Derg",
+ "pos_x": -22.09375,
+ "pos_y": -122.5625,
+ "pos_z": -32.4375,
+ "stations": "Durrance Landing,Hackworth Settlement"
+ },
+ {
+ "name": "Tahademi",
+ "pos_x": 67.75,
+ "pos_y": -6.3125,
+ "pos_z": 145.875,
+ "stations": "Bushnell Orbital,Babcock Dock,Porges Base,Cernan Terminal"
+ },
+ {
+ "name": "Tahu",
+ "pos_x": 17.875,
+ "pos_y": -42.71875,
+ "pos_z": 127.46875,
+ "stations": "Kingsmill Point,Silva Port"
+ },
+ {
+ "name": "Tahuara",
+ "pos_x": 156.21875,
+ "pos_y": -170.78125,
+ "pos_z": 49.09375,
+ "stations": "Urata Dock,Laurent Enterprise"
+ },
+ {
+ "name": "Tahumbla",
+ "pos_x": 63.28125,
+ "pos_y": -95.21875,
+ "pos_z": 122.34375,
+ "stations": "Bracewell Mines,Beshore Mines,Mitchison Silo"
+ },
+ {
+ "name": "Tahuti",
+ "pos_x": 35.03125,
+ "pos_y": -36.5625,
+ "pos_z": -134.75,
+ "stations": "Fernandes de Queiros Dock"
+ },
+ {
+ "name": "Tai He",
+ "pos_x": 90.21875,
+ "pos_y": -259.90625,
+ "pos_z": 9.09375,
+ "stations": "Shinjo Relay,Kobayashi Base,Holden Enterprise"
+ },
+ {
+ "name": "Tai Ho",
+ "pos_x": -41,
+ "pos_y": -2.59375,
+ "pos_z": -160.09375,
+ "stations": "Stefansson Dock,Wallin Hub"
+ },
+ {
+ "name": "Tai Nang Mu",
+ "pos_x": 74.125,
+ "pos_y": -145.25,
+ "pos_z": 137.4375,
+ "stations": "Tan Mines,Walters Vista"
+ },
+ {
+ "name": "Tai Qing",
+ "pos_x": -78.53125,
+ "pos_y": 53.625,
+ "pos_z": 41.40625,
+ "stations": "Soto Station,Celebi Prospect,Dupuy de Lome Vision,Robinson Station"
+ },
+ {
+ "name": "Tai Quambis",
+ "pos_x": -59.09375,
+ "pos_y": -71.78125,
+ "pos_z": 75.5625,
+ "stations": "Alexander Horizons,Silva Horizons,Narvaez Hub,Selye Exchange"
+ },
+ {
+ "name": "Tai Shen",
+ "pos_x": 100.21875,
+ "pos_y": -187.375,
+ "pos_z": -17.1875,
+ "stations": "Parsons Enterprise,Kojima Survey,Bothezat Landing"
+ },
+ {
+ "name": "Tai Shenef",
+ "pos_x": 12.9375,
+ "pos_y": -86.8125,
+ "pos_z": 40.1875,
+ "stations": "Lyne Holdings,Adelman Port"
+ },
+ {
+ "name": "Tai Shere",
+ "pos_x": 73.53125,
+ "pos_y": -51.25,
+ "pos_z": 96.6875,
+ "stations": "Burstein Depot,Fallows Hub,Parkinson Orbital"
+ },
+ {
+ "name": "Tai Sheshy",
+ "pos_x": 214.6875,
+ "pos_y": -124.09375,
+ "pos_z": -3.125,
+ "stations": "Sheremetevsky Dock,Weill Platform,Hammel Ring"
+ },
+ {
+ "name": "Tai Si",
+ "pos_x": -50.03125,
+ "pos_y": 91.15625,
+ "pos_z": 134.9375,
+ "stations": "Sauma Orbital,Witt Depot"
+ },
+ {
+ "name": "Tai Szu",
+ "pos_x": 16.78125,
+ "pos_y": -174.625,
+ "pos_z": 159.46875,
+ "stations": "Kondo Dock,Fremont Settlement,Euthymenes Terminal,Peters Enterprise"
+ },
+ {
+ "name": "Tailgan",
+ "pos_x": 178.0625,
+ "pos_y": -158.71875,
+ "pos_z": 25.53125,
+ "stations": "Coande Platform,Bauman Penal colony"
+ },
+ {
+ "name": "Tailla",
+ "pos_x": -50.40625,
+ "pos_y": 79.6875,
+ "pos_z": -132.59375,
+ "stations": "Lawhead Orbital,Russell Enterprise,Starzl Hub,Sturckow Works"
+ },
+ {
+ "name": "Tailpiri",
+ "pos_x": -104.03125,
+ "pos_y": 149.0625,
+ "pos_z": -9.3125,
+ "stations": "Fanning Ring,Gottlob Frege Enterprise,Schouten City"
+ },
+ {
+ "name": "Tailtsur",
+ "pos_x": 100.40625,
+ "pos_y": -159.71875,
+ "pos_z": 18.15625,
+ "stations": "Phillips Terminal,Naboth Beacon"
+ },
+ {
+ "name": "Taimaiawai",
+ "pos_x": -144.0625,
+ "pos_y": 83.09375,
+ "pos_z": 76.28125,
+ "stations": "Williams Installation,Euclid Hangar"
+ },
+ {
+ "name": "Taimi",
+ "pos_x": -16.3125,
+ "pos_y": -106.78125,
+ "pos_z": 40.34375,
+ "stations": "Perlmutter Prospect,Wilhelm Dock"
+ },
+ {
+ "name": "Taimin",
+ "pos_x": 70.75,
+ "pos_y": 129.59375,
+ "pos_z": 21.96875,
+ "stations": "Slade Ring,Sakers Installation,Cartan's Progress,Kimberlin Vision,Shirley Horizons"
+ },
+ {
+ "name": "Taimyr",
+ "pos_x": -105.84375,
+ "pos_y": 69.1875,
+ "pos_z": 23.03125,
+ "stations": "Edison Relay,Haisheng Camp,Cartan Settlement"
+ },
+ {
+ "name": "Taiocamu",
+ "pos_x": 84.90625,
+ "pos_y": 19.40625,
+ "pos_z": -137.59375,
+ "stations": "Hui Vision,Kagan Enterprise"
+ },
+ {
+ "name": "Taiocha",
+ "pos_x": -94.25,
+ "pos_y": 23.71875,
+ "pos_z": 60.5,
+ "stations": "Koch Stop"
+ },
+ {
+ "name": "Taiowahungu",
+ "pos_x": -122.84375,
+ "pos_y": -39.03125,
+ "pos_z": -58.375,
+ "stations": "Titov City,Schiltberger Depot,Duke Relay"
+ },
+ {
+ "name": "Taiowal",
+ "pos_x": -8.34375,
+ "pos_y": -143.6875,
+ "pos_z": 56.46875,
+ "stations": "Michalitsianos Vision,Woodroffe Station"
+ },
+ {
+ "name": "Taka",
+ "pos_x": -46.28125,
+ "pos_y": 33.96875,
+ "pos_z": -81.625,
+ "stations": "McIntyre Landing"
+ },
+ {
+ "name": "Takak",
+ "pos_x": 127.875,
+ "pos_y": -10.78125,
+ "pos_z": 75.625,
+ "stations": "Filipchenko Vision"
+ },
+ {
+ "name": "Takan",
+ "pos_x": -67.0625,
+ "pos_y": -88.4375,
+ "pos_z": -104.25,
+ "stations": "Karl Diesel Horizons,Voss Relay,Fujimori Barracks"
+ },
+ {
+ "name": "Takshana",
+ "pos_x": 48,
+ "pos_y": -141.125,
+ "pos_z": 4,
+ "stations": "Lee Settlement"
+ },
+ {
+ "name": "Takualmash",
+ "pos_x": -57.59375,
+ "pos_y": 70.625,
+ "pos_z": -110.375,
+ "stations": "Jones Station,Clifford Orbital,Bernoulli Terminal,Card Depot,Andrews Relay"
+ },
+ {
+ "name": "Takurua",
+ "pos_x": -253.8125,
+ "pos_y": -4.0625,
+ "pos_z": -254.1875,
+ "stations": "Foothold Orbital,Mackay's Prospect,Tharp Depot,Stewart Base"
+ },
+ {
+ "name": "Talajie",
+ "pos_x": 57.3125,
+ "pos_y": 167.59375,
+ "pos_z": 36.84375,
+ "stations": "Emshwiller Camp,Flinders Depot"
+ },
+ {
+ "name": "Talantas",
+ "pos_x": -67.78125,
+ "pos_y": 108.6875,
+ "pos_z": 101.3125,
+ "stations": "Scheerbart Orbital,Wrangel Platform,Hoyle Mines,Porges Horizons"
+ },
+ {
+ "name": "Talarhulyms",
+ "pos_x": -61.96875,
+ "pos_y": 18.6875,
+ "pos_z": -133.34375,
+ "stations": "Tryggvason Platform,Smith Beacon"
+ },
+ {
+ "name": "Taleo",
+ "pos_x": -0.40625,
+ "pos_y": 58.34375,
+ "pos_z": -100.46875,
+ "stations": "Le Guin Vision,Lagrange Port"
+ },
+ {
+ "name": "Taliesin",
+ "pos_x": -2.9375,
+ "pos_y": 22.1875,
+ "pos_z": -25.28125,
+ "stations": "Dunbar Platform"
+ },
+ {
+ "name": "Talitha",
+ "pos_x": -5.125,
+ "pos_y": 31.28125,
+ "pos_z": -35.125,
+ "stations": "Jett Station,Tereshkova Gateway,Wakata Gateway,Tuan Station,Stafford Port,Piaget Terminal,Hopkins City,Brady Hub,Gamow City,Wafer Gateway,Solovyev Settlement"
+ },
+ {
+ "name": "Talukana",
+ "pos_x": 119.84375,
+ "pos_y": 80.75,
+ "pos_z": 23.1875,
+ "stations": "Peterson Platform,McDivitt Outpost,Macomb Point"
+ },
+ {
+ "name": "Tama",
+ "pos_x": -29.9375,
+ "pos_y": -45.65625,
+ "pos_z": 10.34375,
+ "stations": "Rzeppa Station,Kafka Arsenal,Hope Port,Arnold Ring,Bierce's Folly"
+ },
+ {
+ "name": "Tamalhikas",
+ "pos_x": 43.1875,
+ "pos_y": -12.15625,
+ "pos_z": -77.4375,
+ "stations": "Horowitz Gateway,Budarin Terminal"
+ },
+ {
+ "name": "Tamali",
+ "pos_x": -110.125,
+ "pos_y": -49.65625,
+ "pos_z": -41.53125,
+ "stations": "Szentmartony Mine,Buckell Dock"
+ },
+ {
+ "name": "Taman",
+ "pos_x": -62.71875,
+ "pos_y": 138.75,
+ "pos_z": -63.90625,
+ "stations": "Evangelisti Sanctuary,Crown Beacon,Cavendish Installation"
+ },
+ {
+ "name": "Tamanaco",
+ "pos_x": 125.25,
+ "pos_y": -120.0625,
+ "pos_z": 90.65625,
+ "stations": "Thomson Port,Hereford Vision"
+ },
+ {
+ "name": "Tamanja",
+ "pos_x": 124.46875,
+ "pos_y": -150.28125,
+ "pos_z": -62.21875,
+ "stations": "Helffrich Stop"
+ },
+ {
+ "name": "Tamanka",
+ "pos_x": 19.84375,
+ "pos_y": -8.15625,
+ "pos_z": 112.875,
+ "stations": "Bean Colony,Hoffman Relay,Mitchison Bastion,Bohm Dock"
+ },
+ {
+ "name": "Tamar",
+ "pos_x": 22.15625,
+ "pos_y": -114.5625,
+ "pos_z": 4.65625,
+ "stations": "Gould Port,Youll City"
+ },
+ {
+ "name": "Tamarilo",
+ "pos_x": 139.125,
+ "pos_y": -115.40625,
+ "pos_z": 103.9375,
+ "stations": "May Ring,Schaumasse Port,Goldreich Vision,Nahavandi Prospect"
+ },
+ {
+ "name": "Tamatz",
+ "pos_x": 143.875,
+ "pos_y": 59.5625,
+ "pos_z": 61.15625,
+ "stations": "Bose Port"
+ },
+ {
+ "name": "Tamaya",
+ "pos_x": -21.4375,
+ "pos_y": -175.25,
+ "pos_z": -20.9375,
+ "stations": "Polikarpov Prospect,Gorgani Platform,Duque Vision"
+ },
+ {
+ "name": "Tamba",
+ "pos_x": 47.59375,
+ "pos_y": -31.96875,
+ "pos_z": 86.46875,
+ "stations": "Khan Beacon"
+ },
+ {
+ "name": "Tambaiamo",
+ "pos_x": 59.21875,
+ "pos_y": 6.25,
+ "pos_z": -58.53125,
+ "stations": "Ayerdhal Refinery,vo Penal colony"
+ },
+ {
+ "name": "Tambe",
+ "pos_x": 20.8125,
+ "pos_y": 78.09375,
+ "pos_z": -40.75,
+ "stations": "McHugh Relay,Efremov Terminal"
+ },
+ {
+ "name": "Tamici",
+ "pos_x": 163.5,
+ "pos_y": -102.53125,
+ "pos_z": 116.875,
+ "stations": "Carrington Hub,Merritt Station"
+ },
+ {
+ "name": "Tamit",
+ "pos_x": -12,
+ "pos_y": -76.5625,
+ "pos_z": -132.3125,
+ "stations": "Irens Dock,Hoshi City,Eudoxus Relay"
+ },
+ {
+ "name": "Tamor",
+ "pos_x": -69.03125,
+ "pos_y": -19.28125,
+ "pos_z": -25.6875,
+ "stations": "Seven Holm,Lerner Survey,Kratman Bastion"
+ },
+ {
+ "name": "Tan'gika",
+ "pos_x": -98.375,
+ "pos_y": -48.96875,
+ "pos_z": -54.625,
+ "stations": "Creighton Refinery"
+ },
+ {
+ "name": "Tan'giko",
+ "pos_x": -1.53125,
+ "pos_y": 26.90625,
+ "pos_z": -157.03125,
+ "stations": "Walters Terminal,Busch Platform"
+ },
+ {
+ "name": "Tan'gunni",
+ "pos_x": -80.1875,
+ "pos_y": 2.90625,
+ "pos_z": 9.5625,
+ "stations": "Low Dock,Creamer Hub,Henslow's Inheritance,Camarda Terminal,Baffin's Inheritance"
+ },
+ {
+ "name": "Tang Mu",
+ "pos_x": 50.03125,
+ "pos_y": -186.0625,
+ "pos_z": 5.46875,
+ "stations": "Kreutz Depot"
+ },
+ {
+ "name": "Tang Warri",
+ "pos_x": -29.25,
+ "pos_y": 92.84375,
+ "pos_z": 49.71875,
+ "stations": "Thurston Installation,Ingstad Hub,Carlisle Base"
+ },
+ {
+ "name": "Tangari",
+ "pos_x": 60.1875,
+ "pos_y": -79.90625,
+ "pos_z": 102.71875,
+ "stations": "Xuesen Beacon,Kimura Landing,Brooks Dock"
+ },
+ {
+ "name": "Tangaroa",
+ "pos_x": -44.8125,
+ "pos_y": -61.6875,
+ "pos_z": -69.6875,
+ "stations": "ASYLUM,Sovica"
+ },
+ {
+ "name": "Tangi",
+ "pos_x": 22.40625,
+ "pos_y": -34.03125,
+ "pos_z": -85.5,
+ "stations": "Wegener's Claim,Dobrovolskiy Prospect"
+ },
+ {
+ "name": "Tangkan",
+ "pos_x": 60.1875,
+ "pos_y": -1.125,
+ "pos_z": 55.875,
+ "stations": "Thomas Base,Alcala Retreat"
+ },
+ {
+ "name": "Tangpho",
+ "pos_x": -96.3125,
+ "pos_y": -30.625,
+ "pos_z": -42.5625,
+ "stations": "Fossum Stop,Agassiz Outpost,Rosenberg Arsenal"
+ },
+ {
+ "name": "Tangphonsa",
+ "pos_x": 129.3125,
+ "pos_y": -79.4375,
+ "pos_z": 103.9375,
+ "stations": "Leuschner Station,De Ring"
+ },
+ {
+ "name": "Tangua",
+ "pos_x": 36.03125,
+ "pos_y": -129.3125,
+ "pos_z": -55.25,
+ "stations": "Wilhelm Orbital,Comper Dock,Navigator Sanctuary,Lange Port,Wallis Orbital,Campbell Depot,Peirce Sanctuary,Freas Port"
+ },
+ {
+ "name": "Tankobung",
+ "pos_x": 46.8125,
+ "pos_y": -176.03125,
+ "pos_z": -81.59375,
+ "stations": "Berkey Terminal,Lockwood Orbital,Leydenfrost Dock,Havilland's Folly"
+ },
+ {
+ "name": "Tanmark",
+ "pos_x": 1.6875,
+ "pos_y": -77.875,
+ "pos_z": -30.09375,
+ "stations": "Cassie-L-Peia,Fernao do Po Dock,Yul Brynner Memorial Station,Parry Market"
+ },
+ {
+ "name": "Tao Dai",
+ "pos_x": 138.4375,
+ "pos_y": -82.75,
+ "pos_z": 106.03125,
+ "stations": "Hulse Dock"
+ },
+ {
+ "name": "Tao Gu",
+ "pos_x": -13.75,
+ "pos_y": -67.6875,
+ "pos_z": 91.90625,
+ "stations": "Wolszczan Station,Koishikawa Port,Puiseux Dock,Abe Plant,Abe Barracks,Struve's Folly"
+ },
+ {
+ "name": "Tao Ti",
+ "pos_x": -1.75,
+ "pos_y": -8.15625,
+ "pos_z": -65.125,
+ "stations": "Schlegel Ring,So-yeon Port,Cavendish Beacon,Chwedyk Hub"
+ },
+ {
+ "name": "Tao Tzaka",
+ "pos_x": -115.625,
+ "pos_y": -22.09375,
+ "pos_z": -99.78125,
+ "stations": "Fossum Plant,Lazarev Terminal,Al Saud's Claim"
+ },
+ {
+ "name": "Tao Wangla",
+ "pos_x": -51.59375,
+ "pos_y": 131.09375,
+ "pos_z": 59.875,
+ "stations": "Norman Enterprise,Roosa Installation,Fuglesang Horizons"
+ },
+ {
+ "name": "Taosha",
+ "pos_x": -113.9375,
+ "pos_y": 18.78125,
+ "pos_z": -10.875,
+ "stations": "Kimberlin Hub,Hugh Hub,Bisson Hub,Margulies Hub,Narbeth Terminal,Schouten Orbital,Jordan Station,Ballard Point,Auld Keep,Sullivan Prospect,Frick Installation,Sabine Installation,Abasheli Depot,Novitski's Claim"
+ },
+ {
+ "name": "Taoshamorio",
+ "pos_x": 157.125,
+ "pos_y": 18.59375,
+ "pos_z": -63.3125,
+ "stations": "Caryanda Orbital,Guerrero Enterprise,Skolem Enterprise,Lichtenberg Penal colony"
+ },
+ {
+ "name": "Taoshan",
+ "pos_x": -87.375,
+ "pos_y": -129.03125,
+ "pos_z": 110.03125,
+ "stations": "Saavedra Dock,Tasman Holdings,Yourkevitch Installation"
+ },
+ {
+ "name": "Taoshas",
+ "pos_x": -99.78125,
+ "pos_y": -0.5,
+ "pos_z": 44,
+ "stations": "Onizuka Prospect"
+ },
+ {
+ "name": "Taotienses",
+ "pos_x": -83.625,
+ "pos_y": 8.28125,
+ "pos_z": 106.4375,
+ "stations": "Burnell's Inheritance,Gamow Retreat,Nyberg Mine"
+ },
+ {
+ "name": "Taotiges",
+ "pos_x": -60.21875,
+ "pos_y": 20.25,
+ "pos_z": 72.28125,
+ "stations": "Yano Terminal,Kimberlin Refinery,Holland Port,Sylvester Settlement"
+ },
+ {
+ "name": "Tapajo",
+ "pos_x": 118.03125,
+ "pos_y": -103.96875,
+ "pos_z": -44.34375,
+ "stations": "Borrelly Gateway,Crown Platform,Bartolomeu de Gusmao Station,Nourse Holdings"
+ },
+ {
+ "name": "Tapany",
+ "pos_x": -21.09375,
+ "pos_y": 79.15625,
+ "pos_z": 147.09375,
+ "stations": "Durrance Works"
+ },
+ {
+ "name": "Tapia",
+ "pos_x": 46.78125,
+ "pos_y": 36.3125,
+ "pos_z": -104.90625,
+ "stations": "Wankel Port,Gerst Platform,Clough's Progress"
+ },
+ {
+ "name": "Tapiete",
+ "pos_x": 149.9375,
+ "pos_y": -72.21875,
+ "pos_z": 54.21875,
+ "stations": "Back Vision,Scotti Hub,Mobius Enterprise,Charlois Orbital,Shepherd Enterprise"
+ },
+ {
+ "name": "Tapirape",
+ "pos_x": 8.15625,
+ "pos_y": -102.65625,
+ "pos_z": 30.15625,
+ "stations": "Inoda Gateway,Schlesinger Terminal,Espenak Port,Fedden Vision"
+ },
+ {
+ "name": "Tapiyan",
+ "pos_x": -117.6875,
+ "pos_y": -91.4375,
+ "pos_z": 4.3125,
+ "stations": "Grant Stop,Bachman Beacon,Clauss Prospect"
+ },
+ {
+ "name": "Tapones",
+ "pos_x": 96.125,
+ "pos_y": -94.75,
+ "pos_z": 80.25,
+ "stations": "Krigstein Vision,Kazantsev Base,Chadwick Observatory"
+ },
+ {
+ "name": "Tapota",
+ "pos_x": 146.03125,
+ "pos_y": 42,
+ "pos_z": -14.875,
+ "stations": "Scheutz Dock,Altman City,Newcomen Survey,Manning Installation,Bunch Base"
+ },
+ {
+ "name": "Tapoya",
+ "pos_x": -154.0625,
+ "pos_y": 28.6875,
+ "pos_z": 79.84375,
+ "stations": "Lander City,Fontenay Terminal,Marusek's Folly"
+ },
+ {
+ "name": "Tara",
+ "pos_x": 7.09375,
+ "pos_y": -9.15625,
+ "pos_z": 41.125,
+ "stations": "Rominger Point,Remek Colony"
+ },
+ {
+ "name": "Tarach Tor",
+ "pos_x": -13.125,
+ "pos_y": -81,
+ "pos_z": -20.28125,
+ "stations": "Carter's Haven,Tranquillity,Russell Prospect"
+ },
+ {
+ "name": "Tarakah",
+ "pos_x": -119.1875,
+ "pos_y": 17.40625,
+ "pos_z": 94.125,
+ "stations": "Evans Port,Rukavishnikov Orbital,Kraepelin Mines,Barentsz Barracks,Mach Camp"
+ },
+ {
+ "name": "Taralurot",
+ "pos_x": 124,
+ "pos_y": -176.96875,
+ "pos_z": -7.09375,
+ "stations": "Chiang Colony,Jordan Plant,Wood Settlement,Penn Holdings"
+ },
+ {
+ "name": "Taramaliwa",
+ "pos_x": 133.46875,
+ "pos_y": -157.09375,
+ "pos_z": 63.25,
+ "stations": "McNaught's Claim,Niemeyer Settlement"
+ },
+ {
+ "name": "Taranis",
+ "pos_x": -52.53125,
+ "pos_y": 18.46875,
+ "pos_z": -28.6875,
+ "stations": "Janszoon Depot"
+ },
+ {
+ "name": "Taras",
+ "pos_x": 100.09375,
+ "pos_y": 55.875,
+ "pos_z": 43.9375,
+ "stations": "Greenland Hub,Schmitz Gateway,Guerrero Beacon"
+ },
+ {
+ "name": "Tarasa",
+ "pos_x": -159.78125,
+ "pos_y": 53.09375,
+ "pos_z": -56.6875,
+ "stations": "Romanenko Platform,Springer Base,Hope Base"
+ },
+ {
+ "name": "Tarbalu",
+ "pos_x": 20.5,
+ "pos_y": -2.6875,
+ "pos_z": -164.1875,
+ "stations": "Aleksandrov Platform"
+ },
+ {
+ "name": "Tarbeh",
+ "pos_x": 156.84375,
+ "pos_y": -180.15625,
+ "pos_z": 16.96875,
+ "stations": "Gehrels Gateway,Blaauw Terminal,Stechkin Landing"
+ },
+ {
+ "name": "Tarbi",
+ "pos_x": -50.15625,
+ "pos_y": 84,
+ "pos_z": -11,
+ "stations": "Farrukh Dock,Kelly Gateway,Alvarado City,Szentmartony Enterprise,Hennepin City,Malchiodi Dock,Isherwood's Progress,Stokes Enterprise"
+ },
+ {
+ "name": "Targamu",
+ "pos_x": -6.78125,
+ "pos_y": -137.34375,
+ "pos_z": -11.65625,
+ "stations": "Barbuy Landing"
+ },
+ {
+ "name": "Targari",
+ "pos_x": -5.53125,
+ "pos_y": -67.1875,
+ "pos_z": 50.3125,
+ "stations": "Hawking Enterprise"
+ },
+ {
+ "name": "Targarku",
+ "pos_x": -54.4375,
+ "pos_y": -1.75,
+ "pos_z": 151,
+ "stations": "Cady Landing,Fu Plant,Pytheas Base"
+ },
+ {
+ "name": "Targk",
+ "pos_x": 38.59375,
+ "pos_y": -103.53125,
+ "pos_z": 99.28125,
+ "stations": "Gustafsson Enterprise,Martin Refinery,Kirshner Survey"
+ },
+ {
+ "name": "Tarhul",
+ "pos_x": 159.5625,
+ "pos_y": 40.625,
+ "pos_z": 1.15625,
+ "stations": "Pashin Ring,Darboux Dock"
+ },
+ {
+ "name": "Taria",
+ "pos_x": 145.03125,
+ "pos_y": -71.09375,
+ "pos_z": -75.40625,
+ "stations": "Parsons Installation,Artzybasheff's Inheritance,Ortiz Moreno Orbital,Liouville Refinery"
+ },
+ {
+ "name": "Tariansa",
+ "pos_x": 150.25,
+ "pos_y": -138.96875,
+ "pos_z": 89.78125,
+ "stations": "Leydenfrost Port"
+ },
+ {
+ "name": "Taribes",
+ "pos_x": -62.3125,
+ "pos_y": -42.75,
+ "pos_z": -62.75,
+ "stations": "Alcala Terminal"
+ },
+ {
+ "name": "Taritra",
+ "pos_x": -20.09375,
+ "pos_y": 80.625,
+ "pos_z": 51.53125,
+ "stations": "Lee Hub,Nicholson Enterprise,Fisk Base"
+ },
+ {
+ "name": "Tariyan",
+ "pos_x": 19.09375,
+ "pos_y": -124.03125,
+ "pos_z": 126,
+ "stations": "Fan Enterprise,Tupolev Terminal,Barreiros Installation"
+ },
+ {
+ "name": "Tartarus",
+ "pos_x": 65.03125,
+ "pos_y": -113.84375,
+ "pos_z": -19.0625,
+ "stations": "Foerster Vision,Gurshtein Colony"
+ },
+ {
+ "name": "Tarude",
+ "pos_x": 63.96875,
+ "pos_y": -35.78125,
+ "pos_z": 15.34375,
+ "stations": "Mendez Orbital,Whitworth Depot,Cabana Platform"
+ },
+ {
+ "name": "Tarup",
+ "pos_x": 121.1875,
+ "pos_y": -60.0625,
+ "pos_z": 48.875,
+ "stations": "Barbuy Survey,Alenquer Installation"
+ },
+ {
+ "name": "Tarutaalli",
+ "pos_x": -73.8125,
+ "pos_y": 21.71875,
+ "pos_z": 40.78125,
+ "stations": "Mayer City,Niven Point"
+ },
+ {
+ "name": "Taruwal",
+ "pos_x": 0.40625,
+ "pos_y": 115.96875,
+ "pos_z": -100.25,
+ "stations": "Patrick Outpost,Lockhart Vision"
+ },
+ {
+ "name": "Tascheter Sector AV-Y b5",
+ "pos_x": -22.5625,
+ "pos_y": -10.03125,
+ "pos_z": -91.5625,
+ "stations": "Detainment Centre Delta-7"
+ },
+ {
+ "name": "Taso Wang",
+ "pos_x": -39.25,
+ "pos_y": -53.3125,
+ "pos_z": 39.40625,
+ "stations": "Nicollier Dock,Thagard Gateway,Velazquez Gateway,Nesvadba Legacy"
+ },
+ {
+ "name": "Taso Wuhte",
+ "pos_x": 96.71875,
+ "pos_y": -112.28125,
+ "pos_z": -19.65625,
+ "stations": "Graham Colony"
+ },
+ {
+ "name": "Tastis",
+ "pos_x": -4.6875,
+ "pos_y": 30.375,
+ "pos_z": -95.96875,
+ "stations": "Ivanishin Platform,Aleksandrov Terminal"
+ },
+ {
+ "name": "Tata",
+ "pos_x": -70.03125,
+ "pos_y": -0.75,
+ "pos_z": 131.90625,
+ "stations": "Wingrove Station,Bulychev Base"
+ },
+ {
+ "name": "Tatamo",
+ "pos_x": 14.65625,
+ "pos_y": 24.65625,
+ "pos_z": 90.34375,
+ "stations": "Spinrad Dock,Gilliland Ring,Plexico Orbital,Weitz Installation,Schmitt Legacy"
+ },
+ {
+ "name": "Tatapai",
+ "pos_x": 57.09375,
+ "pos_y": -56.25,
+ "pos_z": 42.96875,
+ "stations": "Gonnessiat Refinery,Shirazi Estate,Green Vision,Ford's Folly"
+ },
+ {
+ "name": "Tatem",
+ "pos_x": 72.53125,
+ "pos_y": 24.5625,
+ "pos_z": -89.96875,
+ "stations": "Belyayev Ring,von Bellingshausen Landing,Feynman Point"
+ },
+ {
+ "name": "Tati",
+ "pos_x": -120.34375,
+ "pos_y": 35.28125,
+ "pos_z": 105.71875,
+ "stations": "Chasles City,Denton Palace,Cartan Dock,Gottlob Frege Mines,Clifford Depot,Webb Port"
+ },
+ {
+ "name": "Tatiates",
+ "pos_x": -22.21875,
+ "pos_y": 22.90625,
+ "pos_z": 147.8125,
+ "stations": "Banach's Folly,Clifton Survey,al-Khayyam Platform"
+ },
+ {
+ "name": "Tatil",
+ "pos_x": -77.84375,
+ "pos_y": 23.1875,
+ "pos_z": -22.59375,
+ "stations": "Swift Port,Lloyd Terminal,Jacquard Prospect,Roddenberry Dock,Santos Hub,Shepard Ring,Howard Landing,Crampton Installation,Mallory Beacon,Bailey Enterprise,Wood Prospect"
+ },
+ {
+ "name": "Tatjatji",
+ "pos_x": -88.96875,
+ "pos_y": 73.375,
+ "pos_z": 118,
+ "stations": "Apt Beacon,Qurra Outpost"
+ },
+ {
+ "name": "Tatji",
+ "pos_x": 88.75,
+ "pos_y": -14.78125,
+ "pos_z": -95.46875,
+ "stations": "Vinogradov Terminal,Ziemianski Base,Blaschke's Claim"
+ },
+ {
+ "name": "Tatuba",
+ "pos_x": 166.9375,
+ "pos_y": -53.71875,
+ "pos_z": -30.625,
+ "stations": "Boulle Orbital,Bowen Terminal,Iwamoto Barracks,Steinmuller Ring"
+ },
+ {
+ "name": "Tau Bootis",
+ "pos_x": 0.09375,
+ "pos_y": 48.78125,
+ "pos_z": 14.625,
+ "stations": "Schweickart Dock,Nikolayev Terminal,Pascal Orbital,Linteris Dock,Helmholtz City"
+ },
+ {
+ "name": "Tau Centauri",
+ "pos_x": 109.0625,
+ "pos_y": 31.78125,
+ "pos_z": 65.71875,
+ "stations": "Ramelli Gateway,Altman Ring,Lister Port,Searfoss Orbital,Carrier Dock"
+ },
+ {
+ "name": "Tau Ceti",
+ "pos_x": -0.375,
+ "pos_y": -11.40625,
+ "pos_z": -3.5,
+ "stations": "Gilmour Orbiter,Ortiz Moreno City,Graham Terminal,Avogadro Enterprise,Poincare Legacy"
+ },
+ {
+ "name": "Tau Cygni",
+ "pos_x": -65.34375,
+ "pos_y": -8.75,
+ "pos_z": 7.6875,
+ "stations": "Crippen Dock,Kavandi Refinery"
+ },
+ {
+ "name": "Tau Ophiuchii",
+ "pos_x": -19.0625,
+ "pos_y": 6.6875,
+ "pos_z": 52.15625,
+ "stations": "Stokes Terminal,Baker Terminal,Balandin Relay,Robson Hub,Block Hub,Cramer Station,Disch City,Steele Settlement,Utley Park,Holberg Landing"
+ },
+ {
+ "name": "Tau Puppis",
+ "pos_x": 167.8125,
+ "pos_y": -64.34375,
+ "pos_z": -28.75,
+ "stations": "Pogson Vision,Leberecht Tempel Orbital,Sei Survey"
+ },
+ {
+ "name": "Tau Sagittarii",
+ "pos_x": -19.6875,
+ "pos_y": -33.46875,
+ "pos_z": 115.21875,
+ "stations": "Fourneyron Orbital,Kwolek Hub,Hahn Terminal,Burnet Enterprise,Goeppert-Mayer Dock"
+ },
+ {
+ "name": "Tau Sculptoris",
+ "pos_x": 31.6875,
+ "pos_y": -222.3125,
+ "pos_z": -27.1875,
+ "stations": "Witt Prospect,Naylor Beacon"
+ },
+ {
+ "name": "Tau-1 Eridani",
+ "pos_x": 7.75,
+ "pos_y": -40.9375,
+ "pos_z": -20.40625,
+ "stations": "Boabyboy's Barras Station,Killing Time,Tudela Settlement"
+ },
+ {
+ "name": "Tau-1 Gruis",
+ "pos_x": 17.125,
+ "pos_y": -91.4375,
+ "pos_z": 51.59375,
+ "stations": "Kelly Station,Edmondson Dock,Libeskind Port,Nicolet Horizons"
+ },
+ {
+ "name": "Tau-1 Hydrae",
+ "pos_x": 39.59375,
+ "pos_y": 30.96875,
+ "pos_z": -25.84375,
+ "stations": "Priestley Port,Anders Hub,Garratt Orbital,Lehtonen Works"
+ },
+ {
+ "name": "Tau-2 Gruis A",
+ "pos_x": 23.34375,
+ "pos_y": -125.25,
+ "pos_z": 69.8125,
+ "stations": "Apianus Orbital,Kepler Terminal,Thomas Station,Matthews Gateway,Dubyago Station,Mandel Hub,Mattei Vista,Encke Vision,Mattingly Orbital,Konscak Relay,Kranz Terminal,Lintott Hub"
+ },
+ {
+ "name": "Tau-3 Eridani",
+ "pos_x": 24.5625,
+ "pos_y": -76.59375,
+ "pos_z": -37.28125,
+ "stations": "Laveykin Orbital,Musabayev City,Duke Enterprise,Scalzi Vista"
+ },
+ {
+ "name": "Tau-3 Gruis",
+ "pos_x": 37.34375,
+ "pos_y": -210.90625,
+ "pos_z": 115.625,
+ "stations": "Siegel Orbital,Lomonosov Station,Flettner Base"
+ },
+ {
+ "name": "Tauereket",
+ "pos_x": 69,
+ "pos_y": 121.3125,
+ "pos_z": 55.21875,
+ "stations": "Malzberg's Inheritance,Crick Landing,Tryggvason Relay"
+ },
+ {
+ "name": "Tauereks",
+ "pos_x": 71.25,
+ "pos_y": 36,
+ "pos_z": -123.21875,
+ "stations": "Wells Hub,Ford Ring,Bogdanov Station,Szilard Holdings"
+ },
+ {
+ "name": "Taueret",
+ "pos_x": 139.8125,
+ "pos_y": -24.59375,
+ "pos_z": -43.46875,
+ "stations": "Baraniecki Ring,Robson Vision"
+ },
+ {
+ "name": "Tauerni",
+ "pos_x": -67.84375,
+ "pos_y": 7.78125,
+ "pos_z": 60.5625,
+ "stations": "Fincke Dock,Rushworth Port,Scithers Works"
+ },
+ {
+ "name": "Tauerno",
+ "pos_x": -45.78125,
+ "pos_y": 4.40625,
+ "pos_z": -112.625,
+ "stations": "Sturt Dock,Matthews Prospect,Abel Plant"
+ },
+ {
+ "name": "Tauernonese",
+ "pos_x": 43.3125,
+ "pos_y": -61.4375,
+ "pos_z": 12.09375,
+ "stations": "Wakata Port,Galilei Station,Rolland Hub"
+ },
+ {
+ "name": "Tauerritou",
+ "pos_x": -91.25,
+ "pos_y": 132.28125,
+ "pos_z": -26.59375,
+ "stations": "Hennepin Terminal,Normand Settlement,Piccard Prospect"
+ },
+ {
+ "name": "Tauls",
+ "pos_x": -16.4375,
+ "pos_y": 162.15625,
+ "pos_z": -44.1875,
+ "stations": "Conti Reach"
+ },
+ {
+ "name": "Taultam",
+ "pos_x": 104.6875,
+ "pos_y": 72.84375,
+ "pos_z": 36.5625,
+ "stations": "Sabine Estate,Tudela Holdings,Nemere Prospect"
+ },
+ {
+ "name": "Taunetes",
+ "pos_x": -60.9375,
+ "pos_y": -24.125,
+ "pos_z": 72.96875,
+ "stations": "Al Saud Station,Pascal Dock,Carey Terminal,Birmingham Beacon"
+ },
+ {
+ "name": "Taunets",
+ "pos_x": 82.78125,
+ "pos_y": 27.1875,
+ "pos_z": -113.375,
+ "stations": "Forest Works"
+ },
+ {
+ "name": "Tauni",
+ "pos_x": -65.21875,
+ "pos_y": -9.875,
+ "pos_z": -110.5625,
+ "stations": "Dana Orbital,Fung Station"
+ },
+ {
+ "name": "Taunitoq",
+ "pos_x": 186.25,
+ "pos_y": -130.125,
+ "pos_z": 85.6875,
+ "stations": "Royo Horizons,Humason Settlement,Nicholson's Progress"
+ },
+ {
+ "name": "Taurawa",
+ "pos_x": -95.78125,
+ "pos_y": -3.40625,
+ "pos_z": 14.1875,
+ "stations": "Benyovszky Hangar,Svavarsson Landing"
+ },
+ {
+ "name": "Taured",
+ "pos_x": 78.4375,
+ "pos_y": -2.21875,
+ "pos_z": -3.125,
+ "stations": "Duckworth Outpost"
+ },
+ {
+ "name": "Taurina",
+ "pos_x": -56.9375,
+ "pos_y": -77.125,
+ "pos_z": 29.3125,
+ "stations": "Jones Orbital,Norton Orbital,Kerr City,Nomura Beacon,Asire Settlement"
+ },
+ {
+ "name": "Taurinja",
+ "pos_x": 82.53125,
+ "pos_y": 19.65625,
+ "pos_z": -141.6875,
+ "stations": "Laird Landing,Kent Survey"
+ },
+ {
+ "name": "Taurinmuth",
+ "pos_x": -12.53125,
+ "pos_y": -29.59375,
+ "pos_z": 87.09375,
+ "stations": "Jordan Dock,Morris Lab"
+ },
+ {
+ "name": "Taurt",
+ "pos_x": 211.34375,
+ "pos_y": -170.125,
+ "pos_z": 51.84375,
+ "stations": "Froud Enterprise,Koishikawa Station,Eschbach Horizons"
+ },
+ {
+ "name": "Tavgi",
+ "pos_x": 8.34375,
+ "pos_y": -72.84375,
+ "pos_z": 12.84375,
+ "stations": "Gordon Enterprise,Zewail Vision,Ramaswamy Port"
+ },
+ {
+ "name": "Tawaret",
+ "pos_x": 125.84375,
+ "pos_y": -137.75,
+ "pos_z": 38.5,
+ "stations": "Lagadha Plant,Wilson Installation,Lilienthal Hangar,Alexandria Silo"
+ },
+ {
+ "name": "Tawega",
+ "pos_x": -88.03125,
+ "pos_y": 69.8125,
+ "pos_z": 143.59375,
+ "stations": "Heinlein Station,Stefansson City"
+ },
+ {
+ "name": "Tawell",
+ "pos_x": -86.03125,
+ "pos_y": 13.5,
+ "pos_z": -77.03125,
+ "stations": "Robson Ring,Cayley Port,Broderick Enterprise,Macedo Vision,Ings Settlement"
+ },
+ {
+ "name": "Tawembalis",
+ "pos_x": 33.5625,
+ "pos_y": -8.59375,
+ "pos_z": -159.34375,
+ "stations": "Carpenter Settlement,Polyakov Terminal,Lee's Inheritance"
+ },
+ {
+ "name": "Tawembara",
+ "pos_x": 153.5625,
+ "pos_y": -103.59375,
+ "pos_z": 107.15625,
+ "stations": "Kosai Platform,Vess Enterprise,Reamy Hub"
+ },
+ {
+ "name": "Tawer",
+ "pos_x": 117.71875,
+ "pos_y": 37.5625,
+ "pos_z": 75.125,
+ "stations": "Vercors' Claim,Lambert Beacon"
+ },
+ {
+ "name": "Taweret",
+ "pos_x": -16.3125,
+ "pos_y": 136.625,
+ "pos_z": 83.25,
+ "stations": "Parry Platform,Birmingham Horizons,Okorafor Port,Bishop Station,Asher Base"
+ },
+ {
+ "name": "Tawetes",
+ "pos_x": 20.59375,
+ "pos_y": 61.5625,
+ "pos_z": -83.15625,
+ "stations": "Barentsz Plant"
+ },
+ {
+ "name": "Tawi",
+ "pos_x": 42.125,
+ "pos_y": -115.5,
+ "pos_z": -94.125,
+ "stations": "de Kamp Dock,Barnaby Plant,Herschel Hub,Bliss Station,Anthony Enterprise"
+ },
+ {
+ "name": "Tawilo",
+ "pos_x": -25,
+ "pos_y": -96.1875,
+ "pos_z": 81.8125,
+ "stations": "Artin Terminal,Wollheim Port,Brooks Station,Davis Enterprise,Jekhowsky Installation,Anderson Enterprise,Glushko Stop,Saavedra Dock,Cogswell Barracks,Kingsbury Prospect,Warner Orbital,Alexander Orbital,Stjepan Seljan Depot,Napier Terminal"
+ },
+ {
+ "name": "Tawilya",
+ "pos_x": -123.90625,
+ "pos_y": -49.3125,
+ "pos_z": 8.5,
+ "stations": "Salam Legacy,Wilson Retreat"
+ },
+ {
+ "name": "Tawiru",
+ "pos_x": -90.78125,
+ "pos_y": -79.28125,
+ "pos_z": 13.6875,
+ "stations": "Cernan City,Barnwell Enterprise"
+ },
+ {
+ "name": "Tawiskai",
+ "pos_x": 73.25,
+ "pos_y": -113.25,
+ "pos_z": -33.0625,
+ "stations": "Houten-Groeneveld Hub,Soukup Base"
+ },
+ {
+ "name": "Taygeta",
+ "pos_x": -88.5,
+ "pos_y": -159.6875,
+ "pos_z": -366.28125,
+ "stations": "Titan's Daughter"
+ },
+ {
+ "name": "Tche Maini",
+ "pos_x": 74.375,
+ "pos_y": -161.6875,
+ "pos_z": 76.96875,
+ "stations": "Fraknoi Market"
+ },
+ {
+ "name": "Tchehen",
+ "pos_x": 81.90625,
+ "pos_y": 23.8125,
+ "pos_z": -141.28125,
+ "stations": "Ford Dock,Crook Port,Banks Beacon,Laumer Vision,Crown Landing"
+ },
+ {
+ "name": "Tchernobog",
+ "pos_x": 81.96875,
+ "pos_y": 1.21875,
+ "pos_z": 22.03125,
+ "stations": "Vancouver Ring,Lie Base,Shaara Hub,Woolley Orbital,Fabian Beacon,Romanenko Survey"
+ },
+ {
+ "name": "Te Kaha",
+ "pos_x": -172.8125,
+ "pos_y": 13.3125,
+ "pos_z": -154.625,
+ "stations": "New Horizons Orbital"
+ },
+ {
+ "name": "Te Uira",
+ "pos_x": 35.1875,
+ "pos_y": -53.40625,
+ "pos_z": 11.65625,
+ "stations": "Faraday Orbital,Packard Goose,Highbanks"
+ },
+ {
+ "name": "Teaka",
+ "pos_x": -20.65625,
+ "pos_y": -62.28125,
+ "pos_z": 51.875,
+ "stations": "Valdes Dock,Webb Survey,Piercy Terminal,Dedekind City,Ali Legacy,Priest Orbital,MacCurdy City,Akers Market,Mayr Vision,Hutchinson City,Napier Station"
+ },
+ {
+ "name": "Tede",
+ "pos_x": -52.46875,
+ "pos_y": -6.25,
+ "pos_z": 112.40625,
+ "stations": "Lonchakov Hub,Ramelli Holdings,Pook Survey"
+ },
+ {
+ "name": "Tedeinjuna",
+ "pos_x": -67.0625,
+ "pos_y": -179.5,
+ "pos_z": 2.46875,
+ "stations": "Varley Orbital,Woolley Station,Cavendish Port"
+ },
+ {
+ "name": "Tefenhita",
+ "pos_x": -57.8125,
+ "pos_y": 15.59375,
+ "pos_z": 141.34375,
+ "stations": "Tuan Landing,Huygens Relay"
+ },
+ {
+ "name": "Tefenhua",
+ "pos_x": -61.03125,
+ "pos_y": 58.8125,
+ "pos_z": -43.96875,
+ "stations": "Guerrero Orbital,Gilliland Ring,Blish Port,Elder Hub,Doyle Ring,McKee Orbital,Naddoddur City,Silves City,Gromov Orbital,Thornycroft Prospect,Bulgarin Holdings,Conti Installation"
+ },
+ {
+ "name": "Tegidana",
+ "pos_x": -55.03125,
+ "pos_y": -5,
+ "pos_z": -85.75,
+ "stations": "Dickson Settlement,Pawelczyk Depot,Bradshaw Orbital,Ordway Depot,Levi-Civita Hub"
+ },
+ {
+ "name": "Tegiranyan",
+ "pos_x": 24.875,
+ "pos_y": -37.65625,
+ "pos_z": 159.5625,
+ "stations": "Kepler Depot,Currie Survey,Phillips Holdings,Fisher Vision"
+ },
+ {
+ "name": "Tegmen",
+ "pos_x": 31.5625,
+ "pos_y": 35.25,
+ "pos_z": -66.78125,
+ "stations": "Erikson Laboratory,McHugh Bastion,Novitski Station,Singer Terminal,Bohr Orbital"
+ },
+ {
+ "name": "Tehuelche",
+ "pos_x": 135.96875,
+ "pos_y": -187.3125,
+ "pos_z": 37.96875,
+ "stations": "Safdie Port,Drummond Base"
+ },
+ {
+ "name": "Tehuencho",
+ "pos_x": 164.28125,
+ "pos_y": -221.65625,
+ "pos_z": 39.0625,
+ "stations": "van der Riet Woolley Vision,Marriott Colony"
+ },
+ {
+ "name": "Tehuenef",
+ "pos_x": 97.96875,
+ "pos_y": -165.5625,
+ "pos_z": 48.75,
+ "stations": "Mead Station,Jansky Orbital,Moore Ring,Schaumasse Gateway,Martiniere Station,Verne Installation,Danforth Landing,Edmondson Vision"
+ },
+ {
+ "name": "Tehuevi",
+ "pos_x": -76.6875,
+ "pos_y": -119.5625,
+ "pos_z": 40.6875,
+ "stations": "Ford Port,Ray Hub,Haber Hub,Lagrange Keep"
+ },
+ {
+ "name": "Tehuevik",
+ "pos_x": -38.25,
+ "pos_y": 83.5625,
+ "pos_z": -121.4375,
+ "stations": "Farrukh Station"
+ },
+ {
+ "name": "Tehuevisc",
+ "pos_x": 89.9375,
+ "pos_y": -21.8125,
+ "pos_z": 125.625,
+ "stations": "Wylie Port,Ali Depot,Hugh Mine"
+ },
+ {
+ "name": "Tekenatam",
+ "pos_x": -98.3125,
+ "pos_y": 58.5,
+ "pos_z": 101.09375,
+ "stations": "Rayhan al-Biruni Dock,Nicholson Hub,Shiner Dock,Spinrad Base,Schade's Folly"
+ },
+ {
+ "name": "Tekenks",
+ "pos_x": -86.53125,
+ "pos_y": -143.625,
+ "pos_z": -2.25,
+ "stations": "Biggle Vision"
+ },
+ {
+ "name": "Tekkeenat",
+ "pos_x": 111.40625,
+ "pos_y": -124.4375,
+ "pos_z": 130.34375,
+ "stations": "Paul Works"
+ },
+ {
+ "name": "Tekkeener",
+ "pos_x": 33.21875,
+ "pos_y": 149.53125,
+ "pos_z": -67.84375,
+ "stations": "Erdos' Pride,Isherwood Retreat,Vasyutin Terminal"
+ },
+ {
+ "name": "Tekkeenesi",
+ "pos_x": 34.09375,
+ "pos_y": -179.03125,
+ "pos_z": 119.0625,
+ "stations": "Tupolev Vision,O'Neill Prospect,Compton Settlement"
+ },
+ {
+ "name": "Tekkeitjal",
+ "pos_x": 76.5625,
+ "pos_y": 9.34375,
+ "pos_z": -183.4375,
+ "stations": "Riemann Colony,Francisco de Eliza Platform"
+ },
+ {
+ "name": "Telebo",
+ "pos_x": 128.5,
+ "pos_y": 31.46875,
+ "pos_z": -95.21875,
+ "stations": "Herndon Settlement,Birkhoff Base"
+ },
+ {
+ "name": "Teledolyaqa",
+ "pos_x": -25.78125,
+ "pos_y": -123.6875,
+ "pos_z": 27.65625,
+ "stations": "O'Brien Horizons,Abernathy Outpost,Ozanne Prospect"
+ },
+ {
+ "name": "Telengits",
+ "pos_x": 106.09375,
+ "pos_y": -42.125,
+ "pos_z": 117.4375,
+ "stations": "Pike Landing,Abasheli's Progress"
+ },
+ {
+ "name": "Telenisates",
+ "pos_x": 53.03125,
+ "pos_y": 69.84375,
+ "pos_z": 132.1875,
+ "stations": "Atwater Colony,Tull Mines,Gardner Vision"
+ },
+ {
+ "name": "Telente",
+ "pos_x": 181.84375,
+ "pos_y": -110.09375,
+ "pos_z": 48.65625,
+ "stations": "Hatanaka Hub,Shen Port,Serling Base"
+ },
+ {
+ "name": "Telepityak",
+ "pos_x": 19.75,
+ "pos_y": 54.0625,
+ "pos_z": -97.1875,
+ "stations": "Okorafor Relay,Kroehl Orbital,Witt Settlement"
+ },
+ {
+ "name": "Teletes",
+ "pos_x": 139.78125,
+ "pos_y": -184.78125,
+ "pos_z": 65.1875,
+ "stations": "Hind Colony,Bowen Hub,Korolev Mines,Thurston's Folly,Fozard Hub"
+ },
+ {
+ "name": "Teleu",
+ "pos_x": -34.1875,
+ "pos_y": -32.09375,
+ "pos_z": -94.53125,
+ "stations": "Swainson Enterprise,Koch Relay,Maudslay Holdings"
+ },
+ {
+ "name": "Teleut",
+ "pos_x": 74.625,
+ "pos_y": -15.5625,
+ "pos_z": 53.875,
+ "stations": "Grissom Vision,Gold Terminal,H. G. Wells Ring,Crown Dock,Garcia Reach"
+ },
+ {
+ "name": "Televelis",
+ "pos_x": 92.9375,
+ "pos_y": -200.34375,
+ "pos_z": -37.15625,
+ "stations": "Cayley Landing,Chandra Landing,Hatanaka Port"
+ },
+ {
+ "name": "Teliadjali",
+ "pos_x": 109.46875,
+ "pos_y": -79.46875,
+ "pos_z": -38.8125,
+ "stations": "Delsanti Orbital"
+ },
+ {
+ "name": "Telie",
+ "pos_x": 82.5,
+ "pos_y": 50.71875,
+ "pos_z": 4.84375,
+ "stations": "Bujold Vision,Burstein City,Effinger Orbital,Andersson Plant"
+ },
+ {
+ "name": "Telin",
+ "pos_x": 23.71875,
+ "pos_y": -79.4375,
+ "pos_z": -108.71875,
+ "stations": "Heng Station,Melnick Dock,Apgar Orbital,Potrykus Station,Thomas' Claim,Pascal Gateway,Gibson Ring,Volterra Base,Payette City,Garnier Observatory,Klink Refinery,Crown Base"
+ },
+ {
+ "name": "Teliu Yuan",
+ "pos_x": -122.28125,
+ "pos_y": 59.8125,
+ "pos_z": -19.15625,
+ "stations": "Vasilyev Camp,Anthony Mines"
+ },
+ {
+ "name": "Telliocani",
+ "pos_x": 31.6875,
+ "pos_y": -76.28125,
+ "pos_z": 103.75,
+ "stations": "Siddha Port,Beriev Base,Bacigalupi Keep"
+ },
+ {
+ "name": "Telliya",
+ "pos_x": 38.65625,
+ "pos_y": -113.28125,
+ "pos_z": -47.09375,
+ "stations": "Rechtin Station,Ayton Innes Orbital,Palisa Horizons"
+ },
+ {
+ "name": "Tellotait",
+ "pos_x": -38.625,
+ "pos_y": 102.8125,
+ "pos_z": -74.875,
+ "stations": "Beregovoi Outpost"
+ },
+ {
+ "name": "Tellus",
+ "pos_x": 3.34375,
+ "pos_y": 69.28125,
+ "pos_z": -1.65625,
+ "stations": "Ahern Enterprise,Chwedyk Base"
+ },
+ {
+ "name": "Telmarch",
+ "pos_x": 45.59375,
+ "pos_y": 14.75,
+ "pos_z": 67.15625,
+ "stations": "Beliaev Dock,Moore Hangar"
+ },
+ {
+ "name": "Temba",
+ "pos_x": 68.4375,
+ "pos_y": 24.65625,
+ "pos_z": -1.0625,
+ "stations": "Somerset Camp,Xuanzang Point,Howe Holdings"
+ },
+ {
+ "name": "Tembala",
+ "pos_x": -77.34375,
+ "pos_y": -12.21875,
+ "pos_z": 94.21875,
+ "stations": "Parise Station,Thuot Dock,Irvin Port,Bluford Ring,Carrier Gateway,Northrop City,Tesla Terminal,Mohri Port,Pike Settlement,Polya Hub,Bass Works,Schmitt Terminal,Rescue Ship - Parise Station"
+ },
+ {
+ "name": "Temnet",
+ "pos_x": -83.0625,
+ "pos_y": 35,
+ "pos_z": 116.46875,
+ "stations": "Onufrienko Terminal,Clervoy Landing,Harrison Orbital,Parsons Depot"
+ },
+ {
+ "name": "Templatz",
+ "pos_x": -70.34375,
+ "pos_y": -79.9375,
+ "pos_z": 87.125,
+ "stations": "Bethke Vision,Krusvar Vision,Haldeman II Settlement"
+ },
+ {
+ "name": "Temurt",
+ "pos_x": 65.71875,
+ "pos_y": -184.3125,
+ "pos_z": 37.125,
+ "stations": "Jones' Folly,Pritchard Orbital,Littrow Port"
+ },
+ {
+ "name": "Ten Gu",
+ "pos_x": -102.90625,
+ "pos_y": -94.5,
+ "pos_z": 64.71875,
+ "stations": "Cenker Station,Pontes Platform,Kummer Observatory"
+ },
+ {
+ "name": "Ten Mandi",
+ "pos_x": -124.78125,
+ "pos_y": 16.28125,
+ "pos_z": 98.71875,
+ "stations": "Pettit Base,Gernhardt Terminal,Kovalevsky Settlement"
+ },
+ {
+ "name": "Ten Mayan",
+ "pos_x": 143.9375,
+ "pos_y": -198.78125,
+ "pos_z": 105.8125,
+ "stations": "Pilyugin Vision"
+ },
+ {
+ "name": "Ten Samba",
+ "pos_x": 1.9375,
+ "pos_y": -157.8125,
+ "pos_z": -12.3125,
+ "stations": "Webb Port"
+ },
+ {
+ "name": "Tencali",
+ "pos_x": -74.03125,
+ "pos_y": -113,
+ "pos_z": 101.28125,
+ "stations": "Springer Depot"
+ },
+ {
+ "name": "Tenche",
+ "pos_x": -31.125,
+ "pos_y": -74.15625,
+ "pos_z": 37.09375,
+ "stations": "Dixon Vision,Almagro Survey,Maddox's Inheritance,Lavochkin Gateway,Lunney Station,Blaauw Hub,Korolyov Orbital"
+ },
+ {
+ "name": "Tenchih",
+ "pos_x": 111.75,
+ "pos_y": -81.46875,
+ "pos_z": -59.0625,
+ "stations": "Cyrrhus Station,van de Sande Bakhuyzen Vision,Friedman Port,Norman Bastion,Kibalchich Keep,Stapledon Depot"
+ },
+ {
+ "name": "Tenchu",
+ "pos_x": 46.65625,
+ "pos_y": -86.75,
+ "pos_z": -50.84375,
+ "stations": "Franklin Dock"
+ },
+ {
+ "name": "Teng De Di",
+ "pos_x": 4.4375,
+ "pos_y": -20.21875,
+ "pos_z": 109.375,
+ "stations": "Pennington Base,Zhigang Installation,Bohr Depot"
+ },
+ {
+ "name": "Teng Mu",
+ "pos_x": 6.90625,
+ "pos_y": -4.8125,
+ "pos_z": -174.03125,
+ "stations": "Hugh Refinery,Kotzebue Installation"
+ },
+ {
+ "name": "Teng Yeh",
+ "pos_x": 6.28125,
+ "pos_y": 28.4375,
+ "pos_z": -12.46875,
+ "stations": "Morgan Gateway,McBride Station,Brash Hub,Janszoon Depot,Meucci Enterprise,Beregovoi Terminal,Ron Hubbard Terminal,Eisele Orbital,vo Dock,Merbold Port,Whitworth City,Hurston Hub,Sturt Forum"
+ },
+ {
+ "name": "Tengri",
+ "pos_x": 47.03125,
+ "pos_y": -140.0625,
+ "pos_z": -3.25,
+ "stations": "Vic Venables,Guanine Quadruplex"
+ },
+ {
+ "name": "Tengu",
+ "pos_x": 113.28125,
+ "pos_y": 113.6875,
+ "pos_z": -55.78125,
+ "stations": "Ohm Beacon,Swigert Terminal"
+ },
+ {
+ "name": "Tengye",
+ "pos_x": 113.3125,
+ "pos_y": 23.96875,
+ "pos_z": -60.21875,
+ "stations": "Scott Terminal,Braun Orbital,Gauss Port,Roberts' Progress,Malzberg Depot"
+ },
+ {
+ "name": "Tenhar",
+ "pos_x": 113.03125,
+ "pos_y": 31.40625,
+ "pos_z": 20.125,
+ "stations": "Tilman's Claim,Kingsbury's Pride"
+ },
+ {
+ "name": "Tenjin",
+ "pos_x": -9539.4375,
+ "pos_y": -910,
+ "pos_z": 19812.28125,
+ "stations": "Balakor's Research Post"
+ },
+ {
+ "name": "Tenma",
+ "pos_x": -0.5625,
+ "pos_y": -59.4375,
+ "pos_z": 115.75,
+ "stations": "Scobee Dock"
+ },
+ {
+ "name": "Tenmyo",
+ "pos_x": -75.3125,
+ "pos_y": 166.1875,
+ "pos_z": -36.21875,
+ "stations": "al-Khayyam Mine"
+ },
+ {
+ "name": "Teno",
+ "pos_x": 57.28125,
+ "pos_y": -129.9375,
+ "pos_z": 153.40625,
+ "stations": "Asami Landing,Dyr Arena"
+ },
+ {
+ "name": "Tenoi",
+ "pos_x": 171.3125,
+ "pos_y": -133.25,
+ "pos_z": 32.09375,
+ "stations": "Fowler Stop,Lewis Vision"
+ },
+ {
+ "name": "Teorge",
+ "pos_x": 79.4375,
+ "pos_y": 60.65625,
+ "pos_z": 74.3125,
+ "stations": "Conway Survey,Janszoon Station,Sternberg Platform"
+ },
+ {
+ "name": "Tepech",
+ "pos_x": 159,
+ "pos_y": 26.46875,
+ "pos_z": 11.28125,
+ "stations": "Lamarr Enterprise,Hooke Hub,Siemens Port,Spielberg Hub"
+ },
+ {
+ "name": "Tepechua",
+ "pos_x": 118.59375,
+ "pos_y": -149.5625,
+ "pos_z": 33.53125,
+ "stations": "Miller Mines"
+ },
+ {
+ "name": "Tepehuacoc",
+ "pos_x": -13.59375,
+ "pos_y": 69.53125,
+ "pos_z": 63.3125,
+ "stations": "Sauma Orbital,Scheerbart Ring,Anderson City,Shipton Enterprise,Beltrami Forum,Lagrange Port"
+ },
+ {
+ "name": "Tepehuan",
+ "pos_x": 105.65625,
+ "pos_y": -179.9375,
+ "pos_z": 113.375,
+ "stations": "Fairey Works,Ryle Relay,Fan Penal colony"
+ },
+ {
+ "name": "Tepehudti",
+ "pos_x": 79.8125,
+ "pos_y": -135.875,
+ "pos_z": 147.71875,
+ "stations": "Niemeyer Mines,Bohnhoff Relay"
+ },
+ {
+ "name": "Tepehured",
+ "pos_x": 160.25,
+ "pos_y": -77.375,
+ "pos_z": -22.5,
+ "stations": "Druillet Mines"
+ },
+ {
+ "name": "Tepertnyi",
+ "pos_x": -133.4375,
+ "pos_y": 45.625,
+ "pos_z": 92.46875,
+ "stations": "Phillifent Prospect"
+ },
+ {
+ "name": "Tepertsi",
+ "pos_x": 50.21875,
+ "pos_y": -92.59375,
+ "pos_z": 66.09375,
+ "stations": "Zhu City,Flint Point,Zander Dock,Langley Gateway,Pangborn Settlement,Hamilton Reach"
+ },
+ {
+ "name": "Tepi",
+ "pos_x": -2.5625,
+ "pos_y": -121.875,
+ "pos_z": -23.4375,
+ "stations": "Jenkinson Port,Fibonacci Orbital,Szameit Settlement"
+ },
+ {
+ "name": "Tepitara",
+ "pos_x": 175.59375,
+ "pos_y": -154.0625,
+ "pos_z": 19.0625,
+ "stations": "Quetelet Station,Mies van der Rohe Station"
+ },
+ {
+ "name": "Tepitio",
+ "pos_x": 34.8125,
+ "pos_y": -57.21875,
+ "pos_z": -104.5,
+ "stations": "Steele Colony,Hinz Point"
+ },
+ {
+ "name": "Teppani",
+ "pos_x": 8.46875,
+ "pos_y": 170.5625,
+ "pos_z": 27.28125,
+ "stations": "Kinsey Terminal,Edison Port,Marshall Depot,Popov Enterprise"
+ },
+ {
+ "name": "Teppaniach",
+ "pos_x": -111.34375,
+ "pos_y": -30.53125,
+ "pos_z": -40.15625,
+ "stations": "Nusslein-Volhard Ring"
+ },
+ {
+ "name": "Teppanik",
+ "pos_x": -72.28125,
+ "pos_y": -2.75,
+ "pos_z": -115.3125,
+ "stations": "Ohm Terminal,Yeliseyev Orbital,Barsanti Orbital,Roberts Holdings"
+ },
+ {
+ "name": "Teppati",
+ "pos_x": 169.03125,
+ "pos_y": -77.09375,
+ "pos_z": 43.40625,
+ "stations": "Digges Works,VanderMeer Vision"
+ },
+ {
+ "name": "Tereks",
+ "pos_x": -42.125,
+ "pos_y": -121.34375,
+ "pos_z": -3.46875,
+ "stations": "Hill Tinsley Station"
+ },
+ {
+ "name": "Terminus",
+ "pos_x": 81.71875,
+ "pos_y": -6.96875,
+ "pos_z": 25.90625,
+ "stations": "Sanger Orbital,Onizuka Station,Payette Orbital,Wingqvist Reach,Spring Dock,Feoktistov Station,Abel Holdings"
+ },
+ {
+ "name": "Terra Mater",
+ "pos_x": -49.75,
+ "pos_y": -19.03125,
+ "pos_z": -45,
+ "stations": "GR8Minds,Whitelaw Holdings,Chilton Dock,Brendan Dock"
+ },
+ {
+ "name": "Teshub",
+ "pos_x": 3.375,
+ "pos_y": -61.125,
+ "pos_z": -10.78125,
+ "stations": "Brereton Station"
+ },
+ {
+ "name": "Tete",
+ "pos_x": 133.25,
+ "pos_y": -121.90625,
+ "pos_z": -65.34375,
+ "stations": "Hildebrandt's Inheritance,Mattei Dock,Theodor Winnecke Colony,Robert Base,Harper Depot"
+ },
+ {
+ "name": "Tetek",
+ "pos_x": 11.40625,
+ "pos_y": -101.6875,
+ "pos_z": 55.78125,
+ "stations": "Balog Refinery,Pond Hub,Zel'dovich Holdings,Reinmuth Base"
+ },
+ {
+ "name": "Tetekhe",
+ "pos_x": 16.59375,
+ "pos_y": -72.59375,
+ "pos_z": -33.03125,
+ "stations": "Gerst Platform,Goldstein Penal colony"
+ },
+ {
+ "name": "Tetela",
+ "pos_x": 50.53125,
+ "pos_y": -144.78125,
+ "pos_z": 43.25,
+ "stations": "Mueller Orbital,Walotsky's Inheritance,Deslandres Terminal"
+ },
+ {
+ "name": "Tetes",
+ "pos_x": -153.8125,
+ "pos_y": 58.125,
+ "pos_z": 68.6875,
+ "stations": "Pinto Point"
+ },
+ {
+ "name": "Tethlon",
+ "pos_x": 30.1875,
+ "pos_y": 0.09375,
+ "pos_z": 81.46875,
+ "stations": "Mallory Orbital"
+ },
+ {
+ "name": "Tetonang",
+ "pos_x": -89,
+ "pos_y": 29.84375,
+ "pos_z": 98.53125,
+ "stations": "Dalton Orbital,Smith Orbital,Yaping Dock,Ray Port,Newman Hub,Ross Orbital,Lopez de Haro Gateway,Dobzhansky Settlement,Dobrovolski Port"
+ },
+ {
+ "name": "Tetone",
+ "pos_x": 48.53125,
+ "pos_y": -176.09375,
+ "pos_z": 1.875,
+ "stations": "Sunyaev Hub"
+ },
+ {
+ "name": "Tetoneane",
+ "pos_x": -121.78125,
+ "pos_y": -77.5,
+ "pos_z": 117.625,
+ "stations": "Vonnegut Mines"
+ },
+ {
+ "name": "Tetonesir",
+ "pos_x": 0.15625,
+ "pos_y": 134.03125,
+ "pos_z": 43.9375,
+ "stations": "Simak Gateway,Skolem City"
+ },
+ {
+ "name": "Tetrian",
+ "pos_x": 63.125,
+ "pos_y": -50.28125,
+ "pos_z": 38.21875,
+ "stations": "Thanatos Minor,Nicola Station,Eudoxus Dock,Hume Prison"
+ },
+ {
+ "name": "Teurbi",
+ "pos_x": -45.5625,
+ "pos_y": 52.90625,
+ "pos_z": 94.9375,
+ "stations": "Resnick Estate"
+ },
+ {
+ "name": "Teurbolg",
+ "pos_x": -143.25,
+ "pos_y": -63.875,
+ "pos_z": -57.25,
+ "stations": "Herreshoff Survey"
+ },
+ {
+ "name": "Teuricas",
+ "pos_x": 91.40625,
+ "pos_y": -119.71875,
+ "pos_z": 130.34375,
+ "stations": "Asami Mines,Chongzhi Survey,Felice Works"
+ },
+ {
+ "name": "Teushan",
+ "pos_x": -24.46875,
+ "pos_y": 62.25,
+ "pos_z": 116.125,
+ "stations": "Poincare Enterprise,Sutcliffe Dock,Campbell Vision,Morrison Settlement,Bounds Base,Szameit Silo"
+ },
+ {
+ "name": "Teuten",
+ "pos_x": 133.03125,
+ "pos_y": 25.4375,
+ "pos_z": -82.71875,
+ "stations": "Gaspar de Lemos Station,Springer Station,Ford's Progress"
+ },
+ {
+ "name": "Teveri",
+ "pos_x": 65.59375,
+ "pos_y": 58.90625,
+ "pos_z": 85.90625,
+ "stations": "Wiley Port,Brand Hub,Bunsen Hub,Harness Reach,Daniel Settlement"
+ },
+ {
+ "name": "Tewanta",
+ "pos_x": -69.21875,
+ "pos_y": 88.09375,
+ "pos_z": 21.03125,
+ "stations": "Wilson Port,Grimwood Port,Tall Base,Macomb City"
+ },
+ {
+ "name": "Tewi",
+ "pos_x": 79.21875,
+ "pos_y": -112.5625,
+ "pos_z": -4.5625,
+ "stations": "Marcy Vision,Meuron Survey,Baracchi Prospect,Melotte Survey,Cady Depot"
+ },
+ {
+ "name": "Tewi'xilak",
+ "pos_x": -80.03125,
+ "pos_y": -101.15625,
+ "pos_z": 29.71875,
+ "stations": "Zholobov Station,Barba Terminal,Currie Orbital,Newman Terminal,Clifford Terminal,Carrier Terminal,Mach City,Drummond Installation,Padalka Gateway,Deb Orbital,Aubakirov Enterprise,Alvarez de Pineda Legacy,Collins Point,Waldrop Gateway"
+ },
+ {
+ "name": "Tewikwithat",
+ "pos_x": -46.34375,
+ "pos_y": -56.84375,
+ "pos_z": 35.34375,
+ "stations": "Clute Station,Macdonald Station"
+ },
+ {
+ "name": "Tewinicnii",
+ "pos_x": 38.5625,
+ "pos_y": -219.25,
+ "pos_z": -62.15625,
+ "stations": "Meuron Camp"
+ },
+ {
+ "name": "Tewites",
+ "pos_x": 75.46875,
+ "pos_y": -199.28125,
+ "pos_z": -23.875,
+ "stations": "Gorey Relay"
+ },
+ {
+ "name": "Textoverdi",
+ "pos_x": -135.8125,
+ "pos_y": -10.65625,
+ "pos_z": 22.03125,
+ "stations": "de Caminha Survey"
+ },
+ {
+ "name": "Textoveria",
+ "pos_x": -50.5,
+ "pos_y": -137.375,
+ "pos_z": 113.25,
+ "stations": "Britnev Horizons,Taine Hub,McKee Bastion,Anderson Vision"
+ },
+ {
+ "name": "Textovices",
+ "pos_x": 61.78125,
+ "pos_y": -92.0625,
+ "pos_z": 32.8125,
+ "stations": "Bok Hub,Cummings' Folly,Veron Station,Gotz Vision"
+ },
+ {
+ "name": "Thadaguru",
+ "pos_x": 106.15625,
+ "pos_y": 56.78125,
+ "pos_z": 66.9375,
+ "stations": "Bierce Works,Baird Outpost,Bosch's Claim"
+ },
+ {
+ "name": "Thadakha",
+ "pos_x": -18.03125,
+ "pos_y": -190.15625,
+ "pos_z": 73,
+ "stations": "Dhawan Enterprise,Mrkos Terminal,Potagos Vista,Gehry Holdings"
+ },
+ {
+ "name": "Thadandita",
+ "pos_x": 46.53125,
+ "pos_y": -182.125,
+ "pos_z": 48.625,
+ "stations": "Sitterly Colony,Watson Vision"
+ },
+ {
+ "name": "Thaditic",
+ "pos_x": -112.3125,
+ "pos_y": -71.09375,
+ "pos_z": -91.65625,
+ "stations": "Hoyle Estate,Wright Plant"
+ },
+ {
+ "name": "Thadoraton",
+ "pos_x": -101.5,
+ "pos_y": -156.09375,
+ "pos_z": 91.3125,
+ "stations": "Neville Platform"
+ },
+ {
+ "name": "Thadshe",
+ "pos_x": 35.96875,
+ "pos_y": 59.9375,
+ "pos_z": -43.09375,
+ "stations": "Simak Terminal,Brunner Dock,Sekowski Dock,Clapperton Enterprise,Stokes Installation,al-Haytham Hub,Yolen Plant"
+ },
+ {
+ "name": "Thakalaa",
+ "pos_x": -66.28125,
+ "pos_y": -1.96875,
+ "pos_z": 82.03125,
+ "stations": "Kozeyev Relay,Ericsson Camp"
+ },
+ {
+ "name": "Thakan",
+ "pos_x": 134,
+ "pos_y": -242.28125,
+ "pos_z": -20.71875,
+ "stations": "van Houten Colony"
+ },
+ {
+ "name": "Thakpati",
+ "pos_x": -19.0625,
+ "pos_y": 67.78125,
+ "pos_z": 125.90625,
+ "stations": "Martin Reformatory,Hudson Penal colony"
+ },
+ {
+ "name": "Thakur",
+ "pos_x": 81.15625,
+ "pos_y": -161.90625,
+ "pos_z": -83.09375,
+ "stations": "Lindemann Outpost"
+ },
+ {
+ "name": "Thalarctos",
+ "pos_x": 67.0625,
+ "pos_y": -156.28125,
+ "pos_z": -45.78125,
+ "stations": "Elkhome"
+ },
+ {
+ "name": "Thanatos",
+ "pos_x": 80.59375,
+ "pos_y": -74.28125,
+ "pos_z": 12.40625,
+ "stations": "Ibold Works,Amylyn's Rest,Condit's Legacy,Bus Refinery,Holland Point"
+ },
+ {
+ "name": "Tharamamor",
+ "pos_x": -40.53125,
+ "pos_y": -64.5625,
+ "pos_z": 99.71875,
+ "stations": "Preuss Prospect"
+ },
+ {
+ "name": "Thari",
+ "pos_x": 70.9375,
+ "pos_y": -144.6875,
+ "pos_z": -53.28125,
+ "stations": "Jones Orbital,Milne Base,Bassford Dock"
+ },
+ {
+ "name": "Tharni",
+ "pos_x": -66.875,
+ "pos_y": -80.6875,
+ "pos_z": -132.6875,
+ "stations": "Gidzenko's Claim,LeConte Depot,Bean Survey"
+ },
+ {
+ "name": "Tharu",
+ "pos_x": 117.65625,
+ "pos_y": -189.78125,
+ "pos_z": 0.75,
+ "stations": "Wolszczan Orbital,Minkowski Colony,Veron Survey,Luu Mines,Cartan's Inheritance"
+ },
+ {
+ "name": "Tharuburr",
+ "pos_x": -12.78125,
+ "pos_y": -86.15625,
+ "pos_z": 103.59375,
+ "stations": "Wrangell Works"
+ },
+ {
+ "name": "Tharuwa",
+ "pos_x": 73.84375,
+ "pos_y": 31.46875,
+ "pos_z": 160.78125,
+ "stations": "Barnaby Point,Crown Hub"
+ },
+ {
+ "name": "Tharviri",
+ "pos_x": 76.375,
+ "pos_y": -22.53125,
+ "pos_z": -100.59375,
+ "stations": "Lovelace Orbital,Carrasco Plant,Selye Hub,Westerfeld Depot,Fairbairn Orbital"
+ },
+ {
+ "name": "Tharwangu",
+ "pos_x": 5.59375,
+ "pos_y": -20.34375,
+ "pos_z": -97.96875,
+ "stations": "White Mines"
+ },
+ {
+ "name": "Thata",
+ "pos_x": -13.96875,
+ "pos_y": 98.34375,
+ "pos_z": 60.09375,
+ "stations": "Onufriyenko Prospect,Berliner Plant"
+ },
+ {
+ "name": "Thatkwa",
+ "pos_x": 72,
+ "pos_y": -132.125,
+ "pos_z": 159.84375,
+ "stations": "Hoyle Holdings,Fuller Platform"
+ },
+ {
+ "name": "Thauts",
+ "pos_x": 46.46875,
+ "pos_y": -22.5,
+ "pos_z": -128.96875,
+ "stations": "Crippen Orbital,Boulton Platform"
+ },
+ {
+ "name": "Theemetana",
+ "pos_x": 126.8125,
+ "pos_y": 83.90625,
+ "pos_z": -72.75,
+ "stations": "Vries Asylum"
+ },
+ {
+ "name": "Theemim",
+ "pos_x": 125.65625,
+ "pos_y": -139.96875,
+ "pos_z": -101.8125,
+ "stations": "Niemeyer City,Leonard Hub,Dolgov Installation,Crown Arsenal"
+ },
+ {
+ "name": "Thelingi",
+ "pos_x": -33.46875,
+ "pos_y": 138.28125,
+ "pos_z": 93.1875,
+ "stations": "Velho Prospect,Diophantus Holdings,Langsdorff Base,Pinto Prospect"
+ },
+ {
+ "name": "Thelmasci",
+ "pos_x": 45.53125,
+ "pos_y": -117.65625,
+ "pos_z": 61.6875,
+ "stations": "Valz Base,Rolland Vision,Bauschinger Terminal,Dominique Orbital,Burbidge Vision"
+ },
+ {
+ "name": "Themiscrya",
+ "pos_x": 38.3125,
+ "pos_y": -107.875,
+ "pos_z": -6.9375,
+ "stations": "Bamford's Station"
+ },
+ {
+ "name": "Theotokos",
+ "pos_x": 50.125,
+ "pos_y": -49.34375,
+ "pos_z": -20.03125,
+ "stations": "Spring Hangar,Meikle Settlement"
+ },
+ {
+ "name": "Thep No Cha",
+ "pos_x": 67.6875,
+ "pos_y": 28.6875,
+ "pos_z": -74.625,
+ "stations": "Swigert Hub,Garay Terminal,Grover Orbital,Melvill Refinery,Paulmier de Gonneville Landing"
+ },
+ {
+ "name": "Theperigin",
+ "pos_x": 127.875,
+ "pos_y": -70.46875,
+ "pos_z": 111.53125,
+ "stations": "Chretien Oudemans Legacy"
+ },
+ {
+ "name": "Thereila",
+ "pos_x": 41.78125,
+ "pos_y": -58.6875,
+ "pos_z": 87.84375,
+ "stations": "Gann Dock,Payne Holdings,Madsen Colony,Goonan Dock"
+ },
+ {
+ "name": "Thernobo",
+ "pos_x": -30.875,
+ "pos_y": 30.90625,
+ "pos_z": 141.3125,
+ "stations": "Alexeyev Vista,Thoreau Hub,Grabe Hub,Clement Terminal,Lukyanenko Base,Rawat Landing"
+ },
+ {
+ "name": "Theta Capricorni",
+ "pos_x": -67.625,
+ "pos_y": -99.5,
+ "pos_z": 108.78125,
+ "stations": "Lenoir Laboratory,MacDonald Point"
+ },
+ {
+ "name": "Theta Chamaelontis",
+ "pos_x": 134.8125,
+ "pos_y": -58.15625,
+ "pos_z": 50.59375,
+ "stations": "Brom Vision,Shoujing Landing,Matthews Prospect"
+ },
+ {
+ "name": "Theta Indi",
+ "pos_x": 19.84375,
+ "pos_y": -68.46875,
+ "pos_z": 68.375,
+ "stations": "Wyeth City,Vittori's Progress,Stebler Port,Whitney Station,Lefschetz Installation,Levi-Montalcini Ring,Hoffleit's Folly,Conrad Horizons,Barcelos Depot,Fossey Port,Gwynn Vision,Anderson's Progress"
+ },
+ {
+ "name": "Theta Octantis",
+ "pos_x": 133.78125,
+ "pos_y": -139.65625,
+ "pos_z": 98.78125,
+ "stations": "Nouvel Platform"
+ },
+ {
+ "name": "Theta Pavonis",
+ "pos_x": 98.46875,
+ "pos_y": -92.03125,
+ "pos_z": 174.90625,
+ "stations": "Marsden City,Otomo Gateway,Eggleton Hub,Cixin Plant"
+ },
+ {
+ "name": "Theta Phoenicis",
+ "pos_x": 45.4375,
+ "pos_y": -235.71875,
+ "pos_z": 92.40625,
+ "stations": "Asami Stop"
+ },
+ {
+ "name": "Theta Sculptoris",
+ "pos_x": 3.09375,
+ "pos_y": -68.125,
+ "pos_z": 12.96875,
+ "stations": "Hauck Dock,Sadi Carnot Port"
+ },
+ {
+ "name": "Theta Ursae Majoris",
+ "pos_x": -7.59375,
+ "pos_y": 31.71875,
+ "pos_z": -29.46875,
+ "stations": "Bykovsky Terminal,Eyharts Ring,Baxter Base,Merchiston Mine"
+ },
+ {
+ "name": "Theta-1 Microscopii",
+ "pos_x": -3.375,
+ "pos_y": -133.15625,
+ "pos_z": 130.53125,
+ "stations": "Extra Ring,Zhuravleva Vision,Faye City,Tsiolkovsky Ring,Struve Station,Sakers Settlement,Wafer Installation"
+ },
+ {
+ "name": "Thethys",
+ "pos_x": 5.125,
+ "pos_y": -59.75,
+ "pos_z": -56.34375,
+ "stations": "Dantius Citadel of House Thiemann,E.S.S. Owl,Mattingly Settlement,Greenland Depot"
+ },
+ {
+ "name": "Thetis",
+ "pos_x": 75.53125,
+ "pos_y": -25.8125,
+ "pos_z": -22.125,
+ "stations": "Mullane Ring,Cayley Port,Pausch Ring,Johnson Holdings,Nelder Works"
+ },
+ {
+ "name": "Thewi",
+ "pos_x": -19.40625,
+ "pos_y": 13.3125,
+ "pos_z": 145.6875,
+ "stations": "Marlowe Bastion,Pournelle Terminal,Holub Vision,Saberhagen Port"
+ },
+ {
+ "name": "Thewites",
+ "pos_x": 96.28125,
+ "pos_y": -112.46875,
+ "pos_z": 144.9375,
+ "stations": "Zwicky Oasis,Gray Orbital,Lindbohm Depot"
+ },
+ {
+ "name": "Thewixina",
+ "pos_x": 53.4375,
+ "pos_y": -32.25,
+ "pos_z": -36.1875,
+ "stations": "Merbold Terminal,Howard Dock"
+ },
+ {
+ "name": "Thiana",
+ "pos_x": 79.4375,
+ "pos_y": -11.8125,
+ "pos_z": 47.75,
+ "stations": "Gemar Horizons,Yang Port,Kuhn Horizons"
+ },
+ {
+ "name": "Thiansi",
+ "pos_x": -91.21875,
+ "pos_y": 26.40625,
+ "pos_z": 64.875,
+ "stations": "Worlidge Port,Buffett Ring,Newman Ring,Zoline Landing,Seega Base"
+ },
+ {
+ "name": "Thieh",
+ "pos_x": -77.34375,
+ "pos_y": -64.0625,
+ "pos_z": 125.5,
+ "stations": "Eskridge Hub,Beltrami Landing,Person Enterprise"
+ },
+ {
+ "name": "Thiin",
+ "pos_x": 8.75,
+ "pos_y": 18.84375,
+ "pos_z": -71.75,
+ "stations": "Euler Orbital,Brandenstein Enterprise,Dunn Laboratory,Kotov Terminal,Drake Base"
+ },
+ {
+ "name": "Thiini",
+ "pos_x": -37.03125,
+ "pos_y": -17.03125,
+ "pos_z": -117.6875,
+ "stations": "den Berg Stop"
+ },
+ {
+ "name": "Thontar",
+ "pos_x": 170.125,
+ "pos_y": -130.59375,
+ "pos_z": 6.875,
+ "stations": "Jenkins Works"
+ },
+ {
+ "name": "Thor's Helmet Sector FB-X c1-5",
+ "pos_x": 2704.96875,
+ "pos_y": -23.25,
+ "pos_z": -2470.78125,
+ "stations": "Sagan Research Centre"
+ },
+ {
+ "name": "Thos",
+ "pos_x": 108.84375,
+ "pos_y": -76.375,
+ "pos_z": -62.34375,
+ "stations": "Arnold Schwassmann Platform"
+ },
+ {
+ "name": "Thosa",
+ "pos_x": -34.8125,
+ "pos_y": -96.78125,
+ "pos_z": -123.78125,
+ "stations": "Kress Depot"
+ },
+ {
+ "name": "Thosagii",
+ "pos_x": -3.90625,
+ "pos_y": -71.6875,
+ "pos_z": 126.15625,
+ "stations": "Thollon Orbital,Gaultier de Varennes Works,Bauschinger Settlement"
+ },
+ {
+ "name": "Thosangalis",
+ "pos_x": 47.3125,
+ "pos_y": -109.09375,
+ "pos_z": 130.875,
+ "stations": "Seares Survey,Balog Enterprise"
+ },
+ {
+ "name": "Thosia",
+ "pos_x": -77.40625,
+ "pos_y": 101.40625,
+ "pos_z": -61.65625,
+ "stations": "Sagan Orbital,Mach City,Macarthur Orbital,Gresley Station,Guest Terminal,Stott Prospect,Jones Base"
+ },
+ {
+ "name": "Thosiao",
+ "pos_x": -30.25,
+ "pos_y": -86.65625,
+ "pos_z": 93.875,
+ "stations": "Hertzsprung Port,Delporte Market,Keeler Vision,Parker City,Foster Orbital,Flaugergues Orbital,Paulo da Gama Gateway,McCrea Vision"
+ },
+ {
+ "name": "Thosimaki",
+ "pos_x": -21.4375,
+ "pos_y": 129.875,
+ "pos_z": 0.21875,
+ "stations": "Smith Sanctuary,Murphy Prospect"
+ },
+ {
+ "name": "Thosinautas",
+ "pos_x": 133.5625,
+ "pos_y": -142.65625,
+ "pos_z": -20.40625,
+ "stations": "Hussey Point,Thierree Works"
+ },
+ {
+ "name": "Thoth",
+ "pos_x": 1.9375,
+ "pos_y": 41.46875,
+ "pos_z": -24.8125,
+ "stations": "Carpenter Hub,Trevithick Orbital,Hoard Ring,Artsebarsky Terminal,Weston Terminal,England Port,Maire Holdings,Farrer Landing,Polyakov Dock,Nobel Gateway"
+ },
+ {
+ "name": "Thotigudi",
+ "pos_x": -42.03125,
+ "pos_y": -89.90625,
+ "pos_z": 37.625,
+ "stations": "Wiley Port,Herodotus Penal colony"
+ },
+ {
+ "name": "Thotigue",
+ "pos_x": 145.6875,
+ "pos_y": -79.65625,
+ "pos_z": -51.5,
+ "stations": "Fujikawa Mines,Lagadha Installation"
+ },
+ {
+ "name": "Thotti",
+ "pos_x": -70.65625,
+ "pos_y": 88,
+ "pos_z": 107.15625,
+ "stations": "Silves Dock,Lee Dock,Bryant Base"
+ },
+ {
+ "name": "Thra Atius",
+ "pos_x": 20.21875,
+ "pos_y": -23.15625,
+ "pos_z": -159.28125,
+ "stations": "Loncke Landing,Bond Vision"
+ },
+ {
+ "name": "Thra Yax Bard",
+ "pos_x": 143,
+ "pos_y": -83.46875,
+ "pos_z": -37.53125,
+ "stations": "Sutter Colony"
+ },
+ {
+ "name": "Thras",
+ "pos_x": -68.28125,
+ "pos_y": -94.0625,
+ "pos_z": 122.21875,
+ "stations": "Crown Ring,Shonin Station,Ricardo Gateway,Hornby Installation,Crook Horizons,Pavlou Base"
+ },
+ {
+ "name": "Thraskias",
+ "pos_x": 46.71875,
+ "pos_y": 46.625,
+ "pos_z": -1.65625,
+ "stations": "Pettit Ring,Macomb Survey,Willis Stop"
+ },
+ {
+ "name": "Thridan",
+ "pos_x": 99.75,
+ "pos_y": 105.25,
+ "pos_z": 52.90625,
+ "stations": "Rozhdestvensky Enterprise,Sanger Station,Bohm Colony,Alvares' Folly"
+ },
+ {
+ "name": "Thrigpa",
+ "pos_x": 130.59375,
+ "pos_y": 8.21875,
+ "pos_z": -50.3125,
+ "stations": "Ising Platform,Bulleid Relay"
+ },
+ {
+ "name": "Thrimpo",
+ "pos_x": -89.9375,
+ "pos_y": -131.6875,
+ "pos_z": 52.75,
+ "stations": "Nolan Prospect,Noli Installation"
+ },
+ {
+ "name": "Thrite",
+ "pos_x": -11.84375,
+ "pos_y": -108.84375,
+ "pos_z": 84.625,
+ "stations": "Kippax Dock,Sohl Vision,Amundsen Dock,Cassini Installation,Baker Station,Shaver Dock,Elwood Station,Wagner Station,Ellison Prospect,Levinson Station,Gibson Vision"
+ },
+ {
+ "name": "Thrudevashu",
+ "pos_x": 58.90625,
+ "pos_y": -37,
+ "pos_z": 142.71875,
+ "stations": "Cabana Station,Zebrowski Observatory,Metcalf Terminal"
+ },
+ {
+ "name": "Thrudihe",
+ "pos_x": 87.5625,
+ "pos_y": -60.34375,
+ "pos_z": 139.09375,
+ "stations": "Tiptree Terminal,Leinster Prospect"
+ },
+ {
+ "name": "Thruti",
+ "pos_x": 61.625,
+ "pos_y": -126.40625,
+ "pos_z": -98.4375,
+ "stations": "von Biela Holdings,Inoda Platform,Giunta Point,Artsutanov's Claim"
+ },
+ {
+ "name": "Thrutii",
+ "pos_x": -92.46875,
+ "pos_y": -57,
+ "pos_z": 45.5,
+ "stations": "Szentmartony Reach,Butcher Gateway,Mieville Survey,von Krusenstern City"
+ },
+ {
+ "name": "Thrutis",
+ "pos_x": -90.6875,
+ "pos_y": 8.125,
+ "pos_z": -79.53125,
+ "stations": "Kingsbury Dock,Moffitt Enterprise,Baraniecki Arsenal"
+ },
+ {
+ "name": "Thrutti",
+ "pos_x": 124.375,
+ "pos_y": 3.65625,
+ "pos_z": 71.4375,
+ "stations": "Hennepin's Claim"
+ },
+ {
+ "name": "Thule",
+ "pos_x": 27.65625,
+ "pos_y": -131.75,
+ "pos_z": 9.46875,
+ "stations": "Short Hop,Theodorsen Port,Honda Dock"
+ },
+ {
+ "name": "Thunderbird",
+ "pos_x": -3.625,
+ "pos_y": 47.3125,
+ "pos_z": 42.84375,
+ "stations": "Quimby Port,Bischoff Palace,Hawley Port,McCandless Enterprise,Farrer Hub,Shukor Hub,MacLean Dock,Agassiz Orbital,Beregovoi Reach,Stross Camp,Phillpotts Hub,Siemens Enterprise,Dobrovolskiy Hub,Manakov Silo"
+ },
+ {
+ "name": "Ti Wa",
+ "pos_x": -129.8125,
+ "pos_y": -45.625,
+ "pos_z": 1.46875,
+ "stations": "Ingstad City,Hodgkinson Dock,Pratchett Gateway"
+ },
+ {
+ "name": "Ti Wanga",
+ "pos_x": -115.875,
+ "pos_y": -74.96875,
+ "pos_z": 68.59375,
+ "stations": "Roberts Plant,Laird Terminal"
+ },
+ {
+ "name": "Ti Wanjang",
+ "pos_x": 111,
+ "pos_y": -121.53125,
+ "pos_z": 29.3125,
+ "stations": "Somayaji Orbital,Sopwith Forum,Mather Installation"
+ },
+ {
+ "name": "Tialocan",
+ "pos_x": 99.65625,
+ "pos_y": -124.5,
+ "pos_z": -7.71875,
+ "stations": "Dishoeck Terminal,van de Sande Bakhuyzen Station,Theodorsen Hub,Richer Port,Perez Horizons,Somayaji City,Eggen's Folly,Filippenko Settlement,Holland Relay"
+ },
+ {
+ "name": "Tian",
+ "pos_x": 20.5625,
+ "pos_y": -141.21875,
+ "pos_z": 16.75,
+ "stations": "Wallerstein Outpost"
+ },
+ {
+ "name": "Tian Di",
+ "pos_x": 98.84375,
+ "pos_y": 37.875,
+ "pos_z": 43.4375,
+ "stations": "Hornblower Gateway,Cabana Orbital,Ejeta Settlement,Gooch Prospect"
+ },
+ {
+ "name": "Tian Xian",
+ "pos_x": 98.25,
+ "pos_y": 106.40625,
+ "pos_z": 26.40625,
+ "stations": "Ryumin Landing,Mendel City"
+ },
+ {
+ "name": "Tian Yu",
+ "pos_x": 6.15625,
+ "pos_y": -161.90625,
+ "pos_z": 120.78125,
+ "stations": "Lalande Vision,Tombaugh Hub,Le Guin Vision"
+ },
+ {
+ "name": "Tiang K'an",
+ "pos_x": -19.09375,
+ "pos_y": -101.84375,
+ "pos_z": -130.6875,
+ "stations": "Malaspina Colony"
+ },
+ {
+ "name": "Tiang Wu",
+ "pos_x": -47.875,
+ "pos_y": 50.75,
+ "pos_z": 103.1875,
+ "stations": "Barr Landing"
+ },
+ {
+ "name": "Tiangchi",
+ "pos_x": 2.1875,
+ "pos_y": -75.53125,
+ "pos_z": -52.4375,
+ "stations": "Menzies Station,Forstchen Port,Acharya Station,Biggle Landing,Corte-Real City,Vries Port,Roberts Dock,Wylie Port,Hill Orbital,Heaviside Dock,Elder's Claim,Rosseland Settlement"
+ },
+ {
+ "name": "Tiannodaabe",
+ "pos_x": -63.40625,
+ "pos_y": -44.875,
+ "pos_z": 50.375,
+ "stations": "Carver Hangar,Freud Legacy"
+ },
+ {
+ "name": "Tiano",
+ "pos_x": -61.125,
+ "pos_y": -41.5625,
+ "pos_z": -146.4375,
+ "stations": "Burbank Point"
+ },
+ {
+ "name": "Tiansan",
+ "pos_x": -1.4375,
+ "pos_y": 60.46875,
+ "pos_z": -83.75,
+ "stations": "Macgregor Port,Stafford Beacon"
+ },
+ {
+ "name": "Tianve",
+ "pos_x": 90.9375,
+ "pos_y": 31.375,
+ "pos_z": 77.9375,
+ "stations": "Lunan Platform,Baudin Keep"
+ },
+ {
+ "name": "Tiapalan",
+ "pos_x": 26.03125,
+ "pos_y": 18.28125,
+ "pos_z": 9.15625,
+ "stations": "Feoktistov Dock,Eudoxus City,Pasteur Beacon,Ogden Point"
+ },
+ {
+ "name": "Tibi",
+ "pos_x": 205.28125,
+ "pos_y": -140.125,
+ "pos_z": 22.3125,
+ "stations": "Greene Terminal,Wallace Laboratory"
+ },
+ {
+ "name": "Tibila",
+ "pos_x": 139.90625,
+ "pos_y": 41.8125,
+ "pos_z": 0.21875,
+ "stations": "Kuo Gateway,Mayr Orbital,Duckworth Orbital,Nearchus' Folly,Lie Refinery"
+ },
+ {
+ "name": "Tibilja",
+ "pos_x": 147.5,
+ "pos_y": -32.6875,
+ "pos_z": -26.9375,
+ "stations": "Hansteen Works,Oterma Arena"
+ },
+ {
+ "name": "Tibiogamahu",
+ "pos_x": 33.875,
+ "pos_y": -35.625,
+ "pos_z": -124.4375,
+ "stations": "Ohm Depot"
+ },
+ {
+ "name": "Tibis",
+ "pos_x": -34.25,
+ "pos_y": -33.875,
+ "pos_z": 15,
+ "stations": "Sinclair Horizons"
+ },
+ {
+ "name": "Tibitibi",
+ "pos_x": 82.6875,
+ "pos_y": 0.8125,
+ "pos_z": 33.40625,
+ "stations": "Kaku Hub,Torricelli Station,Euler Settlement"
+ },
+ {
+ "name": "Tibitou",
+ "pos_x": 146.59375,
+ "pos_y": -43.3125,
+ "pos_z": 18.125,
+ "stations": "Naboth Station"
+ },
+ {
+ "name": "Tiburi He",
+ "pos_x": -61.3125,
+ "pos_y": -20.90625,
+ "pos_z": -114.65625,
+ "stations": "Milnor Dock,Felice Lab,Pavlou Keep"
+ },
+ {
+ "name": "Tiburnat",
+ "pos_x": 164.84375,
+ "pos_y": -67.28125,
+ "pos_z": 27.75,
+ "stations": "Coye Refinery,Gallun Survey,Kojima City"
+ },
+ {
+ "name": "Ticua",
+ "pos_x": 31.53125,
+ "pos_y": -14.53125,
+ "pos_z": -158.03125,
+ "stations": "Dann Vision,Makarov Orbital,Cayley Lab,Lynn Vision"
+ },
+ {
+ "name": "Ticunchi",
+ "pos_x": -28.875,
+ "pos_y": -134.09375,
+ "pos_z": 80.71875,
+ "stations": "Young Orbital,White Beacon,Whitcomb Plant,Gill Prospect"
+ },
+ {
+ "name": "Ticushpakhi",
+ "pos_x": -67.96875,
+ "pos_y": -42.03125,
+ "pos_z": 19,
+ "stations": "Robinson Point,Ross Hangar,Peral Refinery,Vardeman Platform"
+ },
+ {
+ "name": "Tiegfries",
+ "pos_x": 21.96875,
+ "pos_y": 43,
+ "pos_z": -139,
+ "stations": "Lambert Dock,Garnier Keep,Legendre Dock,Larbalestier Dock"
+ },
+ {
+ "name": "Tiethay",
+ "pos_x": -29.625,
+ "pos_y": 67.09375,
+ "pos_z": -20.875,
+ "stations": "Palmer Platform,Alexeyev Refinery,Baxter Installation"
+ },
+ {
+ "name": "Tiguai",
+ "pos_x": -132.28125,
+ "pos_y": 31.40625,
+ "pos_z": -39.625,
+ "stations": "Pippin Port,Surayev Prospect"
+ },
+ {
+ "name": "Tigue",
+ "pos_x": -101.90625,
+ "pos_y": -49.46875,
+ "pos_z": 106.28125,
+ "stations": "Ehrenfried Kegel Landing,McDevitt Vision"
+ },
+ {
+ "name": "Tigura",
+ "pos_x": -108.8125,
+ "pos_y": 38.5625,
+ "pos_z": 111.15625,
+ "stations": "Isherwood's Exile,Monge Lab"
+ },
+ {
+ "name": "Tigurd",
+ "pos_x": -8.09375,
+ "pos_y": -1.8125,
+ "pos_z": 100.375,
+ "stations": "Savinykh Dock"
+ },
+ {
+ "name": "Tihtipihin",
+ "pos_x": 75.09375,
+ "pos_y": -32.78125,
+ "pos_z": -111.46875,
+ "stations": "Thuot Hub"
+ },
+ {
+ "name": "Tihtir",
+ "pos_x": 90,
+ "pos_y": -63.875,
+ "pos_z": 99.25,
+ "stations": "Tisserand Mines"
+ },
+ {
+ "name": "Tikariyomi",
+ "pos_x": -67.15625,
+ "pos_y": -84.09375,
+ "pos_z": -43.34375,
+ "stations": "Kozlov Dock,Janifer Penal colony"
+ },
+ {
+ "name": "Tikurua",
+ "pos_x": 13.3125,
+ "pos_y": 70.15625,
+ "pos_z": 114.875,
+ "stations": "Bella Port,Gamow Terminal,Ryumin Dock,Khrunov City,Resnik Ring,Gemar Orbital,Haller City,Smith Landing,Cartmill Base"
+ },
+ {
+ "name": "Tiliala",
+ "pos_x": 85.75,
+ "pos_y": -14.09375,
+ "pos_z": -3.625,
+ "stations": "Goldstein High,Dark Star,Brennan Relay,Stein Colony"
+ },
+ {
+ "name": "Tilian",
+ "pos_x": -21.53125,
+ "pos_y": 22.3125,
+ "pos_z": 10.125,
+ "stations": "Maunder's Hope,Keeler Rest,Aleksandrov's Inheritance,Kavandi Depot"
+ },
+ {
+ "name": "Tilo",
+ "pos_x": -53.1875,
+ "pos_y": -99.40625,
+ "pos_z": -0.6875,
+ "stations": "Roosa Landing,Thiele Hub"
+ },
+ {
+ "name": "Timbalderis",
+ "pos_x": 69.09375,
+ "pos_y": 10.03125,
+ "pos_z": 18.40625,
+ "stations": "Roberts Enterprise,Levi-Montalcini Gateway,McKay Terminal,Casper Silo"
+ },
+ {
+ "name": "Timbarichs",
+ "pos_x": 66,
+ "pos_y": -81.03125,
+ "pos_z": 56.5625,
+ "stations": "Vaucouleurs Refinery,Goonan Plant,Fischer Orbital"
+ },
+ {
+ "name": "Timbo",
+ "pos_x": -106.03125,
+ "pos_y": -37.25,
+ "pos_z": -24.96875,
+ "stations": "Drake Reach"
+ },
+ {
+ "name": "Timo Jun",
+ "pos_x": -47.59375,
+ "pos_y": -42.6875,
+ "pos_z": -137.375,
+ "stations": "Knapp Works"
+ },
+ {
+ "name": "Timocan",
+ "pos_x": 88.375,
+ "pos_y": -42.21875,
+ "pos_z": -64.21875,
+ "stations": "Schouten Base,Reamy Mine"
+ },
+ {
+ "name": "Timocani",
+ "pos_x": 24.625,
+ "pos_y": 10.75,
+ "pos_z": -72.40625,
+ "stations": "Pennington Terminal,Seega Port,Maddox Relay"
+ },
+ {
+ "name": "Timoch",
+ "pos_x": 90.15625,
+ "pos_y": 50.46875,
+ "pos_z": -104.34375,
+ "stations": "Agnesi Orbital,Mayr Settlement"
+ },
+ {
+ "name": "Timos",
+ "pos_x": 120.5,
+ "pos_y": -69.1875,
+ "pos_z": -11.21875,
+ "stations": "Hoyle Orbital,Broderick Dock,Kessel Penal colony,von Bellingshausen Station"
+ },
+ {
+ "name": "Tinia",
+ "pos_x": 6.8125,
+ "pos_y": 48.71875,
+ "pos_z": -33.25,
+ "stations": "Jemison Arsenal,Bohm Hub,Levi-Strauss Market,Feynman Beacon,Olivas City"
+ },
+ {
+ "name": "Tinigua",
+ "pos_x": 106.125,
+ "pos_y": -140.6875,
+ "pos_z": 15.5,
+ "stations": "Tomita Ring,Very Orbital,Schaumasse Hub,Gehrels Terminal,Bradfield Orbital,Tsiolkovsky Gateway,Kushida Horizons,Roemer Hub,Friedrich Peters Dock,Goodman Landing,Macdonald Bastion,Kaiser Plant,Uto Port,Corben Vision,Dolgov Landing,Ross Survey"
+ },
+ {
+ "name": "Tiolce",
+ "pos_x": 10.5,
+ "pos_y": -34.78125,
+ "pos_z": 25.0625,
+ "stations": "Gordon Terminal,Cunningham Vision,Malenchenko Dock,Leonov Gateway,Elder Vision,vo Terminal,Grissom Dock,Borisenko Base,Selye Gateway,Laval Settlement,Lawson Gateway,Kwolek Port,Bennett Station,Michelson Enterprise"
+ },
+ {
+ "name": "Tionisla",
+ "pos_x": 82.25,
+ "pos_y": 48.75,
+ "pos_z": 68.15625,
+ "stations": "Brett High,Ing Ring,Coulter City,Kingsmill Camp,Rose Penal colony"
+ },
+ {
+ "name": "Tir",
+ "pos_x": -9532.9375,
+ "pos_y": -923.4375,
+ "pos_z": 19799.125,
+ "stations": "Bolden's Enterprise,Dohler Depot,The Watchtower"
+ },
+ {
+ "name": "Tir Na Nog",
+ "pos_x": 39.5,
+ "pos_y": -95.875,
+ "pos_z": 27.75,
+ "stations": "Pickering Station,Levy Relay,Sabine Keep"
+ },
+ {
+ "name": "Tirada",
+ "pos_x": -56.09375,
+ "pos_y": 55.25,
+ "pos_z": -43.09375,
+ "stations": "Wagner Installation"
+ },
+ {
+ "name": "Tirai",
+ "pos_x": 201.53125,
+ "pos_y": -182.25,
+ "pos_z": 2.15625,
+ "stations": "Laird Arsenal,Apianus Outpost,Coddington Mines"
+ },
+ {
+ "name": "Tirajo",
+ "pos_x": 79.71875,
+ "pos_y": -211.90625,
+ "pos_z": 59.34375,
+ "stations": "Hooker Colony,Newcomb Port,Weyl Enterprise"
+ },
+ {
+ "name": "Tiralla",
+ "pos_x": -7,
+ "pos_y": 15,
+ "pos_z": 88.21875,
+ "stations": "Mastracchio Outpost,Butz Settlement"
+ },
+ {
+ "name": "Tiralya",
+ "pos_x": -122.90625,
+ "pos_y": 63.25,
+ "pos_z": -33.90625,
+ "stations": "Hugh Port,Reed Mines"
+ },
+ {
+ "name": "Tiraocha",
+ "pos_x": 86.75,
+ "pos_y": -70.1875,
+ "pos_z": -84.71875,
+ "stations": "Lopez-Garcia Vision"
+ },
+ {
+ "name": "Tiraon",
+ "pos_x": -86.03125,
+ "pos_y": 30.46875,
+ "pos_z": -138.28125,
+ "stations": "Hippalus Hub,Fadlan Silo,Marley City,Quinn Base"
+ },
+ {
+ "name": "Tirawati",
+ "pos_x": -22.3125,
+ "pos_y": 27.375,
+ "pos_z": 134.59375,
+ "stations": "Andrews Platform,Raleigh Horizons"
+ },
+ {
+ "name": "Tirawishans",
+ "pos_x": -21.65625,
+ "pos_y": -95.9375,
+ "pos_z": -31.5,
+ "stations": "McCoy Port,Linenger Station,Cauchy Relay,Harding Installation"
+ },
+ {
+ "name": "Tirawn",
+ "pos_x": -118.03125,
+ "pos_y": 58.625,
+ "pos_z": 70.5625,
+ "stations": "Bresnik Horizons,Land Mine,Maybury Point"
+ },
+ {
+ "name": "Tirawongla",
+ "pos_x": 80.0625,
+ "pos_y": -99.625,
+ "pos_z": 106.3125,
+ "stations": "Huberath's Folly,Brouwer Terminal,Laurent Enterprise"
+ },
+ {
+ "name": "Tirfing",
+ "pos_x": -65.59375,
+ "pos_y": -1.125,
+ "pos_z": -81.375,
+ "stations": "Tereshkova Settlement,Burgess Base,Murdoch Vision"
+ },
+ {
+ "name": "Tirikohoch",
+ "pos_x": 105.34375,
+ "pos_y": 20.75,
+ "pos_z": 68.53125,
+ "stations": "Cochrane Colony"
+ },
+ {
+ "name": "Tiriku",
+ "pos_x": 93.21875,
+ "pos_y": -86.0625,
+ "pos_z": -68.375,
+ "stations": "Morris Enterprise,Amundsen Vision"
+ },
+ {
+ "name": "Tiris",
+ "pos_x": -26.875,
+ "pos_y": 46.53125,
+ "pos_z": 68.6875,
+ "stations": "Chang-Diaz Orbital,Matteucci Survey,Butler Reach"
+ },
+ {
+ "name": "Titici",
+ "pos_x": -80.125,
+ "pos_y": -120.0625,
+ "pos_z": 96.9375,
+ "stations": "Barnes Mines"
+ },
+ {
+ "name": "Tiveronisa",
+ "pos_x": 89.6875,
+ "pos_y": 28.21875,
+ "pos_z": 58.125,
+ "stations": "Erdos Station,Ozanne Terminal,Watts Orbital,Poincare Station,Whitson Landing,Herbert Port,Almagro Enterprise,Berezin Hub,Gabriel City"
+ },
+ {
+ "name": "Tiverta",
+ "pos_x": 38.6875,
+ "pos_y": 38.125,
+ "pos_z": -119.6875,
+ "stations": "Dezhurov Colony"
+ },
+ {
+ "name": "Tivertsi",
+ "pos_x": -106.6875,
+ "pos_y": 2.03125,
+ "pos_z": -50.0625,
+ "stations": "Forstchen Plant,Francisco de Eliza Prospect,Elwood Colony"
+ },
+ {
+ "name": "Tjakiri",
+ "pos_x": -141.625,
+ "pos_y": -64.375,
+ "pos_z": 59.5625,
+ "stations": "Clair Dock,Simak Landing,Desargues Station,Offutt Dock"
+ },
+ {
+ "name": "Tjakulcade",
+ "pos_x": 52.6875,
+ "pos_y": -78.875,
+ "pos_z": 66,
+ "stations": "Baudin Prospect"
+ },
+ {
+ "name": "Tjal",
+ "pos_x": 142.03125,
+ "pos_y": -62.46875,
+ "pos_z": -73.78125,
+ "stations": "Tsiolkovsky Dock,Howe Oasis,Pimi Terminal"
+ },
+ {
+ "name": "Tjalalang",
+ "pos_x": 68.25,
+ "pos_y": -58.6875,
+ "pos_z": 58.53125,
+ "stations": "Gotz Dock"
+ },
+ {
+ "name": "Tjalan",
+ "pos_x": -38,
+ "pos_y": 4.3125,
+ "pos_z": 161.125,
+ "stations": "Mieville Port,Maybury Depot"
+ },
+ {
+ "name": "Tjalis",
+ "pos_x": 18.375,
+ "pos_y": -81.5625,
+ "pos_z": -79.03125,
+ "stations": "Boe Terminal,Kolmogorov Installation,Gilliland Installation,Herschel Plant"
+ },
+ {
+ "name": "Tjalkond",
+ "pos_x": 45.71875,
+ "pos_y": 74.96875,
+ "pos_z": 73.125,
+ "stations": "Boulton Port,Gibson's Claim"
+ },
+ {
+ "name": "Tjapaho",
+ "pos_x": -66.09375,
+ "pos_y": 64.34375,
+ "pos_z": 128.625,
+ "stations": "Weiss Legacy,Russell Oasis"
+ },
+ {
+ "name": "Tjapai",
+ "pos_x": -34.03125,
+ "pos_y": 67.15625,
+ "pos_z": -112,
+ "stations": "Lee Station,Sverdrup Gateway,Stiegler Orbital,LeConte Terminal,Effinger Dock,Weber Enterprise,Niven Enterprise,Hahn City,Stapledon Ring"
+ },
+ {
+ "name": "Tjapakh",
+ "pos_x": -76.15625,
+ "pos_y": 20,
+ "pos_z": -88.21875,
+ "stations": "Hadamard Dock,Daley Port,Riemann Orbital,McQuay's Progress,Escobar Enterprise,Platt Installation"
+ },
+ {
+ "name": "Tjapakhis",
+ "pos_x": 135.25,
+ "pos_y": -100.21875,
+ "pos_z": 57.9375,
+ "stations": "Baliunas Vision"
+ },
+ {
+ "name": "Tjapan",
+ "pos_x": -32.5625,
+ "pos_y": 37.5,
+ "pos_z": -84.8125,
+ "stations": "Berezovoy Ring,Danvers Barracks,Chilton Dock"
+ },
+ {
+ "name": "Tjapurnaru",
+ "pos_x": -12.90625,
+ "pos_y": 68.34375,
+ "pos_z": -139.46875,
+ "stations": "Regiomontanus Dock,Cooper Landing"
+ },
+ {
+ "name": "Tjeraridjal",
+ "pos_x": 146.09375,
+ "pos_y": -161.625,
+ "pos_z": -33.0625,
+ "stations": "Mil Survey,Dyson Dock,Coppel Works"
+ },
+ {
+ "name": "Tjeraringu",
+ "pos_x": 163.03125,
+ "pos_y": -107.9375,
+ "pos_z": 22.65625,
+ "stations": "Barlowe's Pride"
+ },
+ {
+ "name": "Tjial",
+ "pos_x": -18.78125,
+ "pos_y": 62.8125,
+ "pos_z": 44.4375,
+ "stations": "Jemison City,Cassidy Landing,Mitchell Escape"
+ },
+ {
+ "name": "Tjialfi",
+ "pos_x": -100.6875,
+ "pos_y": 52.15625,
+ "pos_z": -29.125,
+ "stations": "Pashin Landing"
+ },
+ {
+ "name": "Tjialim",
+ "pos_x": 47.03125,
+ "pos_y": -158.84375,
+ "pos_z": -36.15625,
+ "stations": "Gunter Outpost"
+ },
+ {
+ "name": "Tjin",
+ "pos_x": 101.625,
+ "pos_y": -45.59375,
+ "pos_z": -77.71875,
+ "stations": "Pytheas Settlement,Lintott Mines,Ikeya Landing"
+ },
+ {
+ "name": "Tjina",
+ "pos_x": 3.4375,
+ "pos_y": -207.78125,
+ "pos_z": 103.65625,
+ "stations": "Pons' Pride"
+ },
+ {
+ "name": "Tjinawilemi",
+ "pos_x": 61.71875,
+ "pos_y": -18.5625,
+ "pos_z": -135.5625,
+ "stations": "Pierce Platform,Watts Refinery"
+ },
+ {
+ "name": "Tjindjin",
+ "pos_x": 88.28125,
+ "pos_y": 15.65625,
+ "pos_z": 58.40625,
+ "stations": "Cousin Ring,Mitra Keep,Budrys Holdings,Ing Settlement"
+ },
+ {
+ "name": "Tjinggu",
+ "pos_x": -54.90625,
+ "pos_y": 97.09375,
+ "pos_z": 7.5625,
+ "stations": "Miller Survey,Womack Vision,Nixon Base"
+ },
+ {
+ "name": "Tjingola",
+ "pos_x": 61.71875,
+ "pos_y": -59.875,
+ "pos_z": -71.84375,
+ "stations": "Anvil Works,Scalzi Reach,Schouten Vision"
+ },
+ {
+ "name": "Tjinia",
+ "pos_x": 14.75,
+ "pos_y": 70.40625,
+ "pos_z": -146.8125,
+ "stations": "Elwood Dock,Stevin Survey,Hedin Installation"
+ },
+ {
+ "name": "Tjiwan",
+ "pos_x": 163.46875,
+ "pos_y": -96.46875,
+ "pos_z": 93.375,
+ "stations": "Roemer Vision,Axon Horizons"
+ },
+ {
+ "name": "Tjiwang",
+ "pos_x": 125.3125,
+ "pos_y": -7.8125,
+ "pos_z": -86.6875,
+ "stations": "Back Dock,Klein Relay"
+ },
+ {
+ "name": "Tjiwuar",
+ "pos_x": -113.375,
+ "pos_y": 43.53125,
+ "pos_z": 113.75,
+ "stations": "Marques Ring,Felice Station,Pratchett Survey,Hudson Terminal"
+ },
+ {
+ "name": "Tjongxiani",
+ "pos_x": 173.78125,
+ "pos_y": -129.4375,
+ "pos_z": 71.09375,
+ "stations": "Romer Platform,Mason Landing,Zoline Base,Shosuke Penal colony"
+ },
+ {
+ "name": "Tjupa",
+ "pos_x": 113.21875,
+ "pos_y": 22.90625,
+ "pos_z": -92.75,
+ "stations": "McKee Retreat"
+ },
+ {
+ "name": "Tjupalar",
+ "pos_x": 23.75,
+ "pos_y": -236.84375,
+ "pos_z": 80.40625,
+ "stations": "Abbot Depot"
+ },
+ {
+ "name": "Tjupali",
+ "pos_x": 30.9375,
+ "pos_y": -13.75,
+ "pos_z": 172.4375,
+ "stations": "Harrison City,Goeppert-Mayer Orbital,Schweickart Ring,Reightler Holdings,Clifton Vision"
+ },
+ {
+ "name": "Tjupan",
+ "pos_x": 4.5625,
+ "pos_y": -88.09375,
+ "pos_z": 125.75,
+ "stations": "Tsunenaga Port,Payson Base,Wallin Terminal"
+ },
+ {
+ "name": "Tjupanabo",
+ "pos_x": 41.5625,
+ "pos_y": 84.5625,
+ "pos_z": 82.71875,
+ "stations": "Low Orbital,Malocello Station,Tiedemann Installation,Nikitin Station"
+ },
+ {
+ "name": "Tjupany",
+ "pos_x": 19.25,
+ "pos_y": -37.78125,
+ "pos_z": 112.625,
+ "stations": "Wylie Outpost,Goulart Reformatory"
+ },
+ {
+ "name": "Tjurinas",
+ "pos_x": -20.125,
+ "pos_y": -43.71875,
+ "pos_z": 15.40625,
+ "stations": "Napier Terminal,Herndon Dock,Burgess Barracks"
+ },
+ {
+ "name": "Tjurodo",
+ "pos_x": 146.15625,
+ "pos_y": -175.375,
+ "pos_z": 48.78125,
+ "stations": "Zacuto Legacy"
+ },
+ {
+ "name": "Tjurojiu",
+ "pos_x": 26.53125,
+ "pos_y": -76.5,
+ "pos_z": -16.0625,
+ "stations": "Rontgen Enterprise,Oluwafemi Orbital,Volk Settlement,Al-Jazari Station,Zahn Works"
+ },
+ {
+ "name": "Tlak",
+ "pos_x": 137.28125,
+ "pos_y": -103.40625,
+ "pos_z": 50.21875,
+ "stations": "Gulyaev Depot,Giunta Station,Tanaka Horizons,Arrhenius Hub"
+ },
+ {
+ "name": "Tlakakaltan",
+ "pos_x": -7.15625,
+ "pos_y": -141.09375,
+ "pos_z": -1.125,
+ "stations": "Harding Orbital,Lee Landing,Stevin Vision,Ryan Ring,Gill Port"
+ },
+ {
+ "name": "Tlakum",
+ "pos_x": 19.46875,
+ "pos_y": -161.4375,
+ "pos_z": 118.375,
+ "stations": "Anastase Perrotin Gateway,Thierree Port,Cook's Folly,Franke Beacon,Jackson Port"
+ },
+ {
+ "name": "Tlapan",
+ "pos_x": 38.09375,
+ "pos_y": 36.53125,
+ "pos_z": 56.875,
+ "stations": "Ramon Enterprise,Darwin Keep,Onizuka Dock"
+ },
+ {
+ "name": "Tlapana",
+ "pos_x": -21.34375,
+ "pos_y": -89.875,
+ "pos_z": -84.21875,
+ "stations": "Cantor Gateway,Baudin Terminal,Malchiodi Works,Delany Enterprise,King Port,Due Installation,Sladek Port,Bottego Laboratory"
+ },
+ {
+ "name": "Tlapiapo",
+ "pos_x": 47,
+ "pos_y": 21.21875,
+ "pos_z": 119.6875,
+ "stations": "Dobrovolskiy's Inheritance,Bridges Base"
+ },
+ {
+ "name": "Tobala",
+ "pos_x": 23.34375,
+ "pos_y": -42.46875,
+ "pos_z": 79.3125,
+ "stations": "Chun Terminal,Almagro Enterprise,Pratchett Gateway,Lyakhov Base,Conti Point"
+ },
+ {
+ "name": "Tobarci",
+ "pos_x": 18.96875,
+ "pos_y": -20.4375,
+ "pos_z": 117.21875,
+ "stations": "Clifford Colony,Shelley Silo,Taine Mine"
+ },
+ {
+ "name": "Toci",
+ "pos_x": -26.90625,
+ "pos_y": -37.46875,
+ "pos_z": -49.9375,
+ "stations": "Harvest Dock,Lie Hub,Tosi Hide"
+ },
+ {
+ "name": "Toco",
+ "pos_x": 60.875,
+ "pos_y": -101.5,
+ "pos_z": -134.15625,
+ "stations": "Norton Dock"
+ },
+ {
+ "name": "Tocopa",
+ "pos_x": -30.21875,
+ "pos_y": -85.15625,
+ "pos_z": -103.84375,
+ "stations": "Gardner Base"
+ },
+ {
+ "name": "Tocorii",
+ "pos_x": 45.46875,
+ "pos_y": -177.40625,
+ "pos_z": 46.78125,
+ "stations": "Hussey Barracks,Unsold Vision,Oja Port,Smirnova Ring,Cardano Settlement,Moresby Prospect,Danforth Lab"
+ },
+ {
+ "name": "Todzhinas",
+ "pos_x": 64.65625,
+ "pos_y": -52.78125,
+ "pos_z": 150.21875,
+ "stations": "Kimura Terminal,Gutierrez Dock,Apianus Orbital,Boole Beacon"
+ },
+ {
+ "name": "Tofana",
+ "pos_x": -42.96875,
+ "pos_y": 27,
+ "pos_z": -97.5,
+ "stations": "Nelson Ring,Lovell Bastion,Fourier Base"
+ },
+ {
+ "name": "Togher",
+ "pos_x": -16.125,
+ "pos_y": 10.53125,
+ "pos_z": 30.25,
+ "stations": "Scheutz Ring,Bondar Terminal,Lucid Depot,Hurley Park,Brendan Camp"
+ },
+ {
+ "name": "Tojo",
+ "pos_x": 103.875,
+ "pos_y": -181.125,
+ "pos_z": 137.5625,
+ "stations": "Bassford Mine"
+ },
+ {
+ "name": "Toli",
+ "pos_x": 52.875,
+ "pos_y": -74.53125,
+ "pos_z": -87.21875,
+ "stations": "Sudworth Orbital,Lynn Bastion,Cartier Survey"
+ },
+ {
+ "name": "Toli Medeta",
+ "pos_x": -98.125,
+ "pos_y": 102.46875,
+ "pos_z": 87.34375,
+ "stations": "Mastracchio City,Gregory Settlement,Hart Hub,Stableford Enterprise"
+ },
+ {
+ "name": "Tolians",
+ "pos_x": -101.5625,
+ "pos_y": -20.96875,
+ "pos_z": 85.25,
+ "stations": "Kuo Dock,Maudslay Port,Swanson Ring,Stanley Base,Dampier Beacon"
+ },
+ {
+ "name": "Tolini",
+ "pos_x": 42.15625,
+ "pos_y": -53.78125,
+ "pos_z": 145.375,
+ "stations": "Gantt Ring,Frimout Platform,Savinykh Laboratory,Ballard Lab"
+ },
+ {
+ "name": "Tolistshiri",
+ "pos_x": 127.90625,
+ "pos_y": -17.9375,
+ "pos_z": 82.65625,
+ "stations": "McKean Refinery,Salpeter Station,Nesvadba Installation"
+ },
+ {
+ "name": "Tolit",
+ "pos_x": 198.375,
+ "pos_y": -109.375,
+ "pos_z": 29.21875,
+ "stations": "Arrhenius Dock"
+ },
+ {
+ "name": "Tollan",
+ "pos_x": -14.78125,
+ "pos_y": 20,
+ "pos_z": -21.96875,
+ "stations": "Pythagoras Landing,Person Survey,Gordon Plant,MacLean Horizons,Waldeck Keep"
+ },
+ {
+ "name": "Tolmadh",
+ "pos_x": 42.40625,
+ "pos_y": -169.75,
+ "pos_z": -99.75,
+ "stations": "Pearse Point,Porco Prospect"
+ },
+ {
+ "name": "Tolofi",
+ "pos_x": -120.5625,
+ "pos_y": -80.9375,
+ "pos_z": 96.15625,
+ "stations": "Bernoulli Orbital,Rothman Settlement,Reynolds Arsenal"
+ },
+ {
+ "name": "Tolokitan",
+ "pos_x": 97.53125,
+ "pos_y": -81.375,
+ "pos_z": -88.125,
+ "stations": "Carlisle Port,Alvares' Progress,Evans Mines"
+ },
+ {
+ "name": "Tolonese",
+ "pos_x": 135.21875,
+ "pos_y": -110.3125,
+ "pos_z": -49.09375,
+ "stations": "Florine Port,Dixon Terminal,Dickson Horizons,South Dock,Disch Landing"
+ },
+ {
+ "name": "Tolu",
+ "pos_x": 140.09375,
+ "pos_y": -74.78125,
+ "pos_z": 80.15625,
+ "stations": "Kaiser Port,Cuffey Refinery"
+ },
+ {
+ "name": "Toluka",
+ "pos_x": 179.3125,
+ "pos_y": -157.09375,
+ "pos_z": 3.15625,
+ "stations": "Suntzeff Platform"
+ },
+ {
+ "name": "Toluku",
+ "pos_x": 41,
+ "pos_y": 141.59375,
+ "pos_z": 79.96875,
+ "stations": "Foden Orbital,Rotsler Terminal,Crown Lab,Priest Dock"
+ },
+ {
+ "name": "Tolun",
+ "pos_x": 133.4375,
+ "pos_y": -17.125,
+ "pos_z": -68.25,
+ "stations": "Tarentum Vision,Lefschetz Port,Wilkes Enterprise"
+ },
+ {
+ "name": "Tolupan",
+ "pos_x": 162.21875,
+ "pos_y": -3.875,
+ "pos_z": 20.125,
+ "stations": "Anderson Port,Taylor Port,Donaldson Landing,Burton Arsenal"
+ },
+ {
+ "name": "Toma",
+ "pos_x": 84.46875,
+ "pos_y": -218.71875,
+ "pos_z": 62.5,
+ "stations": "Sitter Settlement"
+ },
+ {
+ "name": "Tomani",
+ "pos_x": 61.9375,
+ "pos_y": 87.40625,
+ "pos_z": 44.875,
+ "stations": "Resnick Orbital,Smith Orbital,Gold Dock,Abe Station,Berezin Orbital,Holberg Works,Madsen Reach,Rothman's Progress"
+ },
+ {
+ "name": "Tomas",
+ "pos_x": -15.21875,
+ "pos_y": -28.65625,
+ "pos_z": -64.65625,
+ "stations": "Harris Claim,Willis Settlement"
+ },
+ {
+ "name": "Tomath",
+ "pos_x": -118.75,
+ "pos_y": 97.71875,
+ "pos_z": 17.59375,
+ "stations": "Verrazzano Landing"
+ },
+ {
+ "name": "Tommavas",
+ "pos_x": 46.84375,
+ "pos_y": 109.125,
+ "pos_z": 26.21875,
+ "stations": "Christian City,Manning Installation,Polya Survey,Caselberg's Claim"
+ },
+ {
+ "name": "Tommegin",
+ "pos_x": 53.78125,
+ "pos_y": 83.96875,
+ "pos_z": 60.96875,
+ "stations": "Samokutyayev Hub,Remek Relay"
+ },
+ {
+ "name": "Tommo",
+ "pos_x": 59.6875,
+ "pos_y": -15.09375,
+ "pos_z": -16.21875,
+ "stations": "Hirase Platform,Thiele Vision"
+ },
+ {
+ "name": "Tones",
+ "pos_x": -62.625,
+ "pos_y": 37.375,
+ "pos_z": -63.0625,
+ "stations": "Scott Terminal,Borlaug Landing"
+ },
+ {
+ "name": "Tonesh",
+ "pos_x": 91.59375,
+ "pos_y": -10.5625,
+ "pos_z": -52.125,
+ "stations": "Searfoss Market,Yeliseyev Gateway,Dobrovolski Ring,Satcher Ring,Kimbrough Ring,Houssay Enterprise,Curbeam Prospect,Deb Station"
+ },
+ {
+ "name": "Tonessmeta",
+ "pos_x": 129.3125,
+ "pos_y": 21.84375,
+ "pos_z": 43.8125,
+ "stations": "Malyshev Mine,Coleman Colony"
+ },
+ {
+ "name": "Tong",
+ "pos_x": -102.03125,
+ "pos_y": 15,
+ "pos_z": 35,
+ "stations": "Vela Orbital,Gunn Prospect"
+ },
+ {
+ "name": "Tongaitra",
+ "pos_x": -69.96875,
+ "pos_y": -42.15625,
+ "pos_z": -105.34375,
+ "stations": "Wellman Orbital,Rukavishnikov Market,Aubakirov Enterprise,Bondar Ring,Kregel Enterprise,Kavandi Orbital,Hobaugh Enterprise,Gernhardt Observatory,Grechko Lab,Thurston Depot"
+ },
+ {
+ "name": "Toog",
+ "pos_x": 21.1875,
+ "pos_y": 3.6875,
+ "pos_z": -50.5,
+ "stations": "Bosch Mines,Anders Prospect"
+ },
+ {
+ "name": "Tooges",
+ "pos_x": -129.34375,
+ "pos_y": 6.40625,
+ "pos_z": 108.3125,
+ "stations": "Evans Horizons"
+ },
+ {
+ "name": "Toolfa",
+ "pos_x": 8,
+ "pos_y": -11.1875,
+ "pos_z": -2.65625,
+ "stations": "Crook Hub,Needham Keep,Cady Hub,Monge Keep,Kelleam Orbital"
+ },
+ {
+ "name": "Toor",
+ "pos_x": -87.21875,
+ "pos_y": 6.46875,
+ "pos_z": 30.65625,
+ "stations": "Goetze Meister County [GMC HQ],Deere Settlement"
+ },
+ {
+ "name": "Torenai",
+ "pos_x": -17.65625,
+ "pos_y": 19.9375,
+ "pos_z": -135.90625,
+ "stations": "Grabe Terminal,Herschel Hub,Tuan Station,Panshin Prospect"
+ },
+ {
+ "name": "Toresit",
+ "pos_x": 69.3125,
+ "pos_y": -197.125,
+ "pos_z": 57.1875,
+ "stations": "Chelomey Palace,Bok Orbital,Truly Orbital,Murakami Dock,Merle Settlement,Arrhenius Town,Matthaus Olbers Hub,Bradbury Refinery,Krylov's Progress"
+ },
+ {
+ "name": "Tornakulha",
+ "pos_x": -46.84375,
+ "pos_y": -88.90625,
+ "pos_z": 68.53125,
+ "stations": "Yolen Sanctuary"
+ },
+ {
+ "name": "Torngala",
+ "pos_x": -115.375,
+ "pos_y": 40.3125,
+ "pos_z": -109.9375,
+ "stations": "Bessemer Settlement,Cameron Outpost,Byrd Landing"
+ },
+ {
+ "name": "Torngita",
+ "pos_x": -0.9375,
+ "pos_y": 37.71875,
+ "pos_z": 86.96875,
+ "stations": "Burnet Ring,Alas Terminal,Cameron Dock"
+ },
+ {
+ "name": "Torno",
+ "pos_x": -134.15625,
+ "pos_y": -34.4375,
+ "pos_z": -116.09375,
+ "stations": "Gardner Port,Young Dock,Coelho Hub,Pinzon Holdings,Wandrei Beacon"
+ },
+ {
+ "name": "Toro",
+ "pos_x": -71.28125,
+ "pos_y": -103,
+ "pos_z": -19.375,
+ "stations": "Adams' Folly,Faris Dock"
+ },
+ {
+ "name": "Toroesing",
+ "pos_x": -70.78125,
+ "pos_y": -85.53125,
+ "pos_z": -87.71875,
+ "stations": "Ciferri Dock,Clifford Port,Kurland Installation,Reilly Dock,Hornblower Base,Coulomb Orbital,Ryazanski Vision"
+ },
+ {
+ "name": "Toromona",
+ "pos_x": -40.1875,
+ "pos_y": 33.75,
+ "pos_z": 24.9375,
+ "stations": "Lorenz Station"
+ },
+ {
+ "name": "Tosaka",
+ "pos_x": 17.40625,
+ "pos_y": -157.125,
+ "pos_z": -99.9375,
+ "stations": "Greene Port,Islam Platform"
+ },
+ {
+ "name": "Tosakanth",
+ "pos_x": 36.65625,
+ "pos_y": 68.25,
+ "pos_z": -66.15625,
+ "stations": "Boe Platform"
+ },
+ {
+ "name": "Tote",
+ "pos_x": -21.0625,
+ "pos_y": -15.75,
+ "pos_z": -91.75,
+ "stations": "Whitelaw Enterprise,Carrasco Settlement,Tucker Penal colony"
+ },
+ {
+ "name": "Totegafook",
+ "pos_x": 142.65625,
+ "pos_y": 57.21875,
+ "pos_z": -43.625,
+ "stations": "Garn Outpost"
+ },
+ {
+ "name": "Totenis",
+ "pos_x": 189.5625,
+ "pos_y": -122.9375,
+ "pos_z": 74.21875,
+ "stations": "Pippin Survey,Edwards Terminal"
+ },
+ {
+ "name": "Totjob'al",
+ "pos_x": -77.375,
+ "pos_y": -13.5625,
+ "pos_z": -81.3125,
+ "stations": "Vishweswarayya Plant,Henry Hangar"
+ },
+ {
+ "name": "Tougei",
+ "pos_x": -24.03125,
+ "pos_y": 123.28125,
+ "pos_z": -113.25,
+ "stations": "Richards Port,Land Plant,Taylor Silo"
+ },
+ {
+ "name": "Tougeir",
+ "pos_x": -81.6875,
+ "pos_y": 42.53125,
+ "pos_z": -83.625,
+ "stations": "Mallory Dock,Scortia Dock,Janes Dock"
+ },
+ {
+ "name": "Tougeirs",
+ "pos_x": 27.3125,
+ "pos_y": -34.75,
+ "pos_z": -43.90625,
+ "stations": "Dobrovolski City,Boswell Orbital,Walter Survey"
+ },
+ {
+ "name": "Toxand",
+ "pos_x": 85,
+ "pos_y": -11.25,
+ "pos_z": 60.625,
+ "stations": "Furukawa Hub,Viehbock Orbital,Le Guin Base,Garriott Exchange,Cardano Station"
+ },
+ {
+ "name": "Toxanda",
+ "pos_x": -32.59375,
+ "pos_y": 34.96875,
+ "pos_z": -89.625,
+ "stations": "Key Settlement,Preuss' Inheritance"
+ },
+ {
+ "name": "Toxandh",
+ "pos_x": 119.5625,
+ "pos_y": -166.46875,
+ "pos_z": 42.5,
+ "stations": "Jackson Settlement,Bartlett Arsenal,Carnera Base"
+ },
+ {
+ "name": "Toxandji",
+ "pos_x": 155.09375,
+ "pos_y": -12.40625,
+ "pos_z": 61.0625,
+ "stations": "Tsunenaga Orbital,Alvares Dock,Bohm Landing,Morrow Port,Williams Survey"
+ },
+ {
+ "name": "Toyeri",
+ "pos_x": 49.1875,
+ "pos_y": -215.78125,
+ "pos_z": 39.875,
+ "stations": "Lagerkvist Base"
+ },
+ {
+ "name": "Toyony",
+ "pos_x": 74.71875,
+ "pos_y": -1.84375,
+ "pos_z": -79.75,
+ "stations": "Dukaj Depot,Powell Mine"
+ },
+ {
+ "name": "Toyopa",
+ "pos_x": 155.625,
+ "pos_y": -38.28125,
+ "pos_z": 33.78125,
+ "stations": "King Orbital,Giles Horizons,Schomburg Silo"
+ },
+ {
+ "name": "Tpheim",
+ "pos_x": 55.625,
+ "pos_y": -223.125,
+ "pos_z": 79.34375,
+ "stations": "MacCready Mine"
+ },
+ {
+ "name": "Tpheirset",
+ "pos_x": -37.46875,
+ "pos_y": 67.5,
+ "pos_z": 97.59375,
+ "stations": "Nordenskiold Ring,Kandel Orbital,Haldeman Depot,Gottlob Frege Ring,Tilley Camp,Boulle Point,Birkhoff Vision,Clifford Base"
+ },
+ {
+ "name": "Tphen Slata",
+ "pos_x": 77.625,
+ "pos_y": 44.5,
+ "pos_z": -63.3125,
+ "stations": "Hassanein Station,McCarthy Station,Windt Enterprise,Doyle Depot,Nehsi Works"
+ },
+ {
+ "name": "Tphene",
+ "pos_x": -94.28125,
+ "pos_y": -39.21875,
+ "pos_z": 67.25,
+ "stations": "Hamilton Depot"
+ },
+ {
+ "name": "Trakath",
+ "pos_x": -9534.78125,
+ "pos_y": -908.96875,
+ "pos_z": 19798.9375,
+ "stations": "Diva Mines,Safe Haven"
+ },
+ {
+ "name": "Trani",
+ "pos_x": 87.53125,
+ "pos_y": -72.40625,
+ "pos_z": 110.21875,
+ "stations": "Borrelly Port,Loewy Silo"
+ },
+ {
+ "name": "Trante",
+ "pos_x": 128.875,
+ "pos_y": -3.5,
+ "pos_z": 26.40625,
+ "stations": "Ewald Platform,Flinders Lab,Tokarev Plant,Sohl Depot"
+ },
+ {
+ "name": "Trany",
+ "pos_x": -92.53125,
+ "pos_y": 80.4375,
+ "pos_z": 79.875,
+ "stations": "Battuta Relay"
+ },
+ {
+ "name": "Tranyuwa",
+ "pos_x": -9.875,
+ "pos_y": -152.21875,
+ "pos_z": -70.84375,
+ "stations": "Land Hub,Vinogradov Beacon,Clauss Relay"
+ },
+ {
+ "name": "Treima",
+ "pos_x": -37,
+ "pos_y": -114,
+ "pos_z": 65,
+ "stations": "Mooz's Hope,Bisnovatyi-Kogan Depot"
+ },
+ {
+ "name": "Trella",
+ "pos_x": -57.375,
+ "pos_y": 14.59375,
+ "pos_z": 60.625,
+ "stations": "Tito Colony"
+ },
+ {
+ "name": "Trepin",
+ "pos_x": 26.375,
+ "pos_y": 10.5625,
+ "pos_z": 9.78125,
+ "stations": "Rukavishnikov Hub,Menzies' Inheritance,Gaffney Ring,Kuhn Hub,Khrunov Survey"
+ },
+ {
+ "name": "Trevakat",
+ "pos_x": -130.90625,
+ "pos_y": -11.625,
+ "pos_z": 83.4375,
+ "stations": "Palmer Vision,Poisson Enterprise,Tokubei Vision,Quick Works"
+ },
+ {
+ "name": "Trianguli Sector EQ-X a1-1",
+ "pos_x": 91.5625,
+ "pos_y": -43.09375,
+ "pos_z": -114.6875,
+ "stations": "Miletus Landing"
+ },
+ {
+ "name": "Trianguli Sector FL-Y c6",
+ "pos_x": 97.75,
+ "pos_y": -34.40625,
+ "pos_z": -104.1875,
+ "stations": "Kopra Silo,Caidin Vision"
+ },
+ {
+ "name": "Trianguli Sector GG-Y c18",
+ "pos_x": 72.1875,
+ "pos_y": -66.625,
+ "pos_z": -103.3125,
+ "stations": "Maybury Enterprise"
+ },
+ {
+ "name": "Tribe",
+ "pos_x": 190.65625,
+ "pos_y": -124.34375,
+ "pos_z": 8.8125,
+ "stations": "Delporte Hub,Vavrova Enterprise,Richer Vision"
+ },
+ {
+ "name": "Tribeb",
+ "pos_x": 111.625,
+ "pos_y": -55.96875,
+ "pos_z": -57.40625,
+ "stations": "Antonio de Andrade Plant,Huggins Mines"
+ },
+ {
+ "name": "Trica",
+ "pos_x": 148.375,
+ "pos_y": 63.78125,
+ "pos_z": -63.46875,
+ "stations": "Bosch Enterprise,Aubakirov Relay,Linteris Silo"
+ },
+ {
+ "name": "Tricasses",
+ "pos_x": 167.5625,
+ "pos_y": -130.96875,
+ "pos_z": -27.25,
+ "stations": "Westerhout City,Lavochkin Ring,Iwamoto Vision,Stromgren's Folly,Banks Landing"
+ },
+ {
+ "name": "Tricastini",
+ "pos_x": 133.375,
+ "pos_y": 36.5625,
+ "pos_z": 10,
+ "stations": "Stross Port"
+ },
+ {
+ "name": "Tricates",
+ "pos_x": -16.625,
+ "pos_y": 85.5625,
+ "pos_z": 148.375,
+ "stations": "Underwood Stop"
+ },
+ {
+ "name": "Tricor",
+ "pos_x": 71.5625,
+ "pos_y": -19.5625,
+ "pos_z": -35.0625,
+ "stations": "Morukov Gateway,Ballard Arsenal,Elvstrom Base"
+ },
+ {
+ "name": "Tricorii",
+ "pos_x": -31.34375,
+ "pos_y": 72,
+ "pos_z": 99.84375,
+ "stations": "Hippalus Orbital,Ross Gateway,McAllaster Hub,Gottlob Frege Base,Paulmier de Gonneville Settlement"
+ },
+ {
+ "name": "Trifid Sector IR-W d1-52",
+ "pos_x": -612.40625,
+ "pos_y": -31.5,
+ "pos_z": 5182.875,
+ "stations": "Observation Post Epsilon"
+ },
+ {
+ "name": "Trigen",
+ "pos_x": 6.59375,
+ "pos_y": 142.78125,
+ "pos_z": -69.9375,
+ "stations": "Stefansson Dock,Cartan Terminal,Gerlache Camp"
+ },
+ {
+ "name": "Trigeno",
+ "pos_x": 74.53125,
+ "pos_y": -82.71875,
+ "pos_z": 121.5,
+ "stations": "Hansen Port,Tuttle Terminal,Calatrava Dock,Taylor Colony,Baille Base,Baillaud Settlement"
+ },
+ {
+ "name": "Trigosan",
+ "pos_x": -37,
+ "pos_y": -112.6875,
+ "pos_z": 82.90625,
+ "stations": "Savery Settlement,Kooi Port,Grimwood Bastion"
+ },
+ {
+ "name": "Trimairusa",
+ "pos_x": -133.3125,
+ "pos_y": -28.875,
+ "pos_z": 79.75,
+ "stations": "Anthony de la Roche Outpost,Galiano Penal colony"
+ },
+ {
+ "name": "Trimarindji",
+ "pos_x": 66.46875,
+ "pos_y": -83.5625,
+ "pos_z": 117.125,
+ "stations": "Dawes Station,Kneale's Folly,Zeppelin Survey,Tange Dock,Yuzhe Port"
+ },
+ {
+ "name": "Trimir",
+ "pos_x": 23.25,
+ "pos_y": -1.6875,
+ "pos_z": 110.625,
+ "stations": "Whitney Station,Stanier Terminal,Murray Port,Malyshev Station,Higginbotham City,Reed Works,Lenthall Vision"
+ },
+ {
+ "name": "Trinar",
+ "pos_x": 49.75,
+ "pos_y": 104.78125,
+ "pos_z": 70.375,
+ "stations": "McClintock Terminal,Joliot-Curie Enterprise,Bulgarin Plant,Dunbar Beacon,Wellman Dock"
+ },
+ {
+ "name": "Tring",
+ "pos_x": -130.34375,
+ "pos_y": -6.03125,
+ "pos_z": -52.0625,
+ "stations": "Fischer Port,Usachov Hub,Lopez-Alegria Station,Gibson City,Ross Station,LeConte Landing,Parise Depot,Poindexter Depot"
+ },
+ {
+ "name": "Trinifex",
+ "pos_x": 68.8125,
+ "pos_y": 7.375,
+ "pos_z": 157.59375,
+ "stations": "Turtledove Orbital,Tevis Point"
+ },
+ {
+ "name": "Tripitaka",
+ "pos_x": 58.375,
+ "pos_y": 9.71875,
+ "pos_z": 80.90625,
+ "stations": "Liwei Terminal,Hale Gateway,Wundt City,Dezhurov Hub,Velazquez's Claim"
+ },
+ {
+ "name": "Tripu",
+ "pos_x": -73.15625,
+ "pos_y": 9.28125,
+ "pos_z": -27.8125,
+ "stations": "Titov Orbital,Leonov Arsenal,Chargaff Orbital,Cormack Vision,Scott Enterprise"
+ },
+ {
+ "name": "Tripuna",
+ "pos_x": -62.0625,
+ "pos_y": -73.6875,
+ "pos_z": -47.0625,
+ "stations": "Noli Enterprise,Adams Dock,Rucker Enterprise,King Holdings"
+ },
+ {
+ "name": "Tripuri",
+ "pos_x": 159.375,
+ "pos_y": -116.03125,
+ "pos_z": 49.71875,
+ "stations": "Baille Settlement,van Vogt Hub"
+ },
+ {
+ "name": "Tripuru",
+ "pos_x": 28.71875,
+ "pos_y": 5.1875,
+ "pos_z": -128.1875,
+ "stations": "Nagata Beacon,Drew Dock,Gohar Refinery"
+ },
+ {
+ "name": "Tripurus",
+ "pos_x": 104.40625,
+ "pos_y": -179.1875,
+ "pos_z": 140.9375,
+ "stations": "Abel's Folly,Proctor Oasis,Hague Prospect"
+ },
+ {
+ "name": "Triquagsak",
+ "pos_x": -71.875,
+ "pos_y": -118.15625,
+ "pos_z": 35.5625,
+ "stations": "Walker Relay,Werber Beacon,Pauli Port"
+ },
+ {
+ "name": "TrishiGaut",
+ "pos_x": 51.1875,
+ "pos_y": 80.84375,
+ "pos_z": -33.40625,
+ "stations": "Mukai Market,Clifton Point,Schlegel Terminal,Buchli Survey,Hand Escape,Barnes Enterprise,Herreshoff Enterprise"
+ },
+ {
+ "name": "Triteia",
+ "pos_x": -47.3125,
+ "pos_y": 16.90625,
+ "pos_z": -65.90625,
+ "stations": "Hopkins Gateway"
+ },
+ {
+ "name": "Triton",
+ "pos_x": 67.75,
+ "pos_y": -43.15625,
+ "pos_z": -21.5,
+ "stations": "Shepard Orbital,White Terminal,Brady Station,Wang Terminal"
+ },
+ {
+ "name": "Triviatii",
+ "pos_x": 70.75,
+ "pos_y": -26.6875,
+ "pos_z": -96.21875,
+ "stations": "Carpini Colony,Hilbert Refinery"
+ },
+ {
+ "name": "Troch",
+ "pos_x": -5,
+ "pos_y": -225.125,
+ "pos_z": -27.0625,
+ "stations": "Foerster Camp"
+ },
+ {
+ "name": "Trociti",
+ "pos_x": 33.21875,
+ "pos_y": 47.625,
+ "pos_z": 79.3125,
+ "stations": "Arrhenius Relay,Collins Platform"
+ },
+ {
+ "name": "Trocmii",
+ "pos_x": 88.28125,
+ "pos_y": -180.6875,
+ "pos_z": -23.34375,
+ "stations": "Kolmogorov Dock,Gwynn Port,Abe Arsenal,Chandler Hub,Ellern Base"
+ },
+ {
+ "name": "Trocnades",
+ "pos_x": -25.46875,
+ "pos_y": -32.40625,
+ "pos_z": 21.5,
+ "stations": "Franklin Hub,Coulomb Platform,Reynolds Depot"
+ },
+ {
+ "name": "Trones",
+ "pos_x": 121.125,
+ "pos_y": 126.25,
+ "pos_z": 22,
+ "stations": "Hartog Outpost"
+ },
+ {
+ "name": "Trong",
+ "pos_x": 86.8125,
+ "pos_y": -33.78125,
+ "pos_z": 57.09375,
+ "stations": "Searfoss Vision"
+ },
+ {
+ "name": "Trongbanza",
+ "pos_x": 25.625,
+ "pos_y": -114.96875,
+ "pos_z": 98.09375,
+ "stations": "Orbik Vision,Froud Orbital,Riazuddin Exchange,Wul Terminal,Balog Dock,Barnwell Vision"
+ },
+ {
+ "name": "Trua",
+ "pos_x": -87.46875,
+ "pos_y": 12.9375,
+ "pos_z": -38.3125,
+ "stations": "Walz Platform"
+ },
+ {
+ "name": "Truk",
+ "pos_x": -23,
+ "pos_y": -143.71875,
+ "pos_z": 100.0625,
+ "stations": "Wenzel Gateway"
+ },
+ {
+ "name": "Trukampasuk",
+ "pos_x": 64.40625,
+ "pos_y": -18.21875,
+ "pos_z": 11.5,
+ "stations": "Lie Station,Hasse Relay,Bradley Settlement"
+ },
+ {
+ "name": "Truku",
+ "pos_x": -59.90625,
+ "pos_y": -3.1875,
+ "pos_z": 135.84375,
+ "stations": "Tucker Vision"
+ },
+ {
+ "name": "Trukulha",
+ "pos_x": -31.84375,
+ "pos_y": 107.625,
+ "pos_z": 86.59375,
+ "stations": "Fiennes Dock,Geston Stop"
+ },
+ {
+ "name": "Trumanuchu",
+ "pos_x": -2.875,
+ "pos_y": 36.875,
+ "pos_z": 58.4375,
+ "stations": "Manarov Enterprise,Vian Enterprise,Khayyam Dock,Springer Market"
+ },
+ {
+ "name": "Trumbo",
+ "pos_x": 110.9375,
+ "pos_y": -55.5,
+ "pos_z": 90.875,
+ "stations": "Burgess Installation,Gent Oasis,Mikoyan Platform"
+ },
+ {
+ "name": "Trumnito",
+ "pos_x": -68.15625,
+ "pos_y": -183,
+ "pos_z": -25.28125,
+ "stations": "Conti Survey"
+ },
+ {
+ "name": "Trumuye",
+ "pos_x": 96.59375,
+ "pos_y": -46.125,
+ "pos_z": -11.6875,
+ "stations": "Yakovlev Port,d'Allonville Dock,Foerster Port"
+ },
+ {
+ "name": "Ts'ai Shai",
+ "pos_x": -16.5,
+ "pos_y": -101.625,
+ "pos_z": 128.6875,
+ "stations": "Leberecht Tempel Port"
+ },
+ {
+ "name": "Ts'ai Yukou",
+ "pos_x": 92.3125,
+ "pos_y": -137.78125,
+ "pos_z": -108.875,
+ "stations": "Gunter Landing,Husband Enterprise"
+ },
+ {
+ "name": "Ts'ao Gong",
+ "pos_x": 99.3125,
+ "pos_y": 43.9375,
+ "pos_z": -97.9375,
+ "stations": "Houtman Platform,Schiltberger Refinery"
+ },
+ {
+ "name": "Ts'ao Hii",
+ "pos_x": -80.53125,
+ "pos_y": -13.9375,
+ "pos_z": 102.03125,
+ "stations": "Stanier Hub,Cassidy Gateway,Rashid City,Allen Port,Garneau Dock,Alexander Base,Boole Relay,Willis Enterprise"
+ },
+ {
+ "name": "Ts'ao Tach",
+ "pos_x": 112.25,
+ "pos_y": 66.65625,
+ "pos_z": 20.6875,
+ "stations": "Coulter Vision,Malchiodi Plant,Beltrami Terminal"
+ },
+ {
+ "name": "Ts'in",
+ "pos_x": 54.21875,
+ "pos_y": 71,
+ "pos_z": 142.21875,
+ "stations": "Detmer Base,Chretien Hub,Hughes Oasis"
+ },
+ {
+ "name": "Ts'in Gu",
+ "pos_x": -116.1875,
+ "pos_y": -47.71875,
+ "pos_z": 41.96875,
+ "stations": "Nelson Port,Stirling's Inheritance,Wandrei Arsenal,Moon Installation"
+ },
+ {
+ "name": "Ts'in Yanka",
+ "pos_x": 86.53125,
+ "pos_y": -3.46875,
+ "pos_z": -87.3125,
+ "stations": "Linge Gateway,Wallace Station,Hiraga Gateway"
+ },
+ {
+ "name": "Tsak",
+ "pos_x": 9.34375,
+ "pos_y": -136.71875,
+ "pos_z": 98.65625,
+ "stations": "Alphonsi Station,Naylor Port,Rothfuss Vision"
+ },
+ {
+ "name": "Tsakapai",
+ "pos_x": 148.4375,
+ "pos_y": -263.90625,
+ "pos_z": 66.875,
+ "stations": "Alden Reformatory,McKee Mines"
+ },
+ {
+ "name": "Tsakhmet",
+ "pos_x": 124.5,
+ "pos_y": 47.65625,
+ "pos_z": 127.6875,
+ "stations": "Hill Dock,Person's Folly,Kelly Landing,Alvares Colony,Brust Point,Aldiss Works"
+ },
+ {
+ "name": "Tsanas",
+ "pos_x": 73.0625,
+ "pos_y": 28.03125,
+ "pos_z": -111.78125,
+ "stations": "Bova Arena,Christopher Relay,Daley Survey"
+ },
+ {
+ "name": "Tsanla",
+ "pos_x": 61.75,
+ "pos_y": -159.09375,
+ "pos_z": 116.59375,
+ "stations": "Huss Dock,Schmidt Station,Littrow Dock,de Balboa Exchange"
+ },
+ {
+ "name": "Tsanluo Wu",
+ "pos_x": -88.09375,
+ "pos_y": -62.40625,
+ "pos_z": 64.1875,
+ "stations": "Deere Dock,Gamow Dock,Ashby Relay,Brillant Beacon"
+ },
+ {
+ "name": "Tsanyala",
+ "pos_x": 43.34375,
+ "pos_y": -109.25,
+ "pos_z": 84.09375,
+ "stations": "Endate Vision,Stokes Base,Fehrenbach Bastion"
+ },
+ {
+ "name": "Tsao Chun",
+ "pos_x": -153.8125,
+ "pos_y": -70.875,
+ "pos_z": -24.9375,
+ "stations": "Piaget Installation,Thomas Point"
+ },
+ {
+ "name": "Tsao Wang",
+ "pos_x": 64.15625,
+ "pos_y": 7.15625,
+ "pos_z": 118.9375,
+ "stations": "Budarin Terminal,Spinrad Landing,Yang Gateway,Tull Ring,Lem Barracks"
+ },
+ {
+ "name": "Tschapa",
+ "pos_x": 139.03125,
+ "pos_y": -169.875,
+ "pos_z": 33.78125,
+ "stations": "Fraknoi Hub,Shea Vision,d'Allonville Bastion"
+ },
+ {
+ "name": "Tsche",
+ "pos_x": 1.46875,
+ "pos_y": -66.875,
+ "pos_z": 133.28125,
+ "stations": "Macan Lab,Seares Escape,Makarov Hangar,Velho Hub"
+ },
+ {
+ "name": "Tse",
+ "pos_x": 136.375,
+ "pos_y": 54.625,
+ "pos_z": 41.78125,
+ "stations": "Bresnik Mine,Hennen Point"
+ },
+ {
+ "name": "Tsetan",
+ "pos_x": -112.75,
+ "pos_y": 17.71875,
+ "pos_z": -34.71875,
+ "stations": "Heck Refinery,Sheckley Relay,Hippalus Settlement"
+ },
+ {
+ "name": "Tsetiaci",
+ "pos_x": 161,
+ "pos_y": -14.5,
+ "pos_z": 82.46875,
+ "stations": "Garfinkle Mines,Coblentz Survey,Elgin Colony"
+ },
+ {
+ "name": "Tsetsegei",
+ "pos_x": 62.125,
+ "pos_y": -26.21875,
+ "pos_z": 42.53125,
+ "stations": "Bykovsky Colony,Tarelkin Base"
+ },
+ {
+ "name": "Tsetsi",
+ "pos_x": 163.0625,
+ "pos_y": -31.03125,
+ "pos_z": -45.1875,
+ "stations": "Fontana Installation,Thiele Settlement"
+ },
+ {
+ "name": "Tsetyerre",
+ "pos_x": -17.9375,
+ "pos_y": 135.96875,
+ "pos_z": 63,
+ "stations": "Farrer Orbital,Jett Ring,Pryor Bastion,Normand Relay"
+ },
+ {
+ "name": "Tsim Biko",
+ "pos_x": 37.28125,
+ "pos_y": 158.5625,
+ "pos_z": -35.84375,
+ "stations": "Evans Dock,Laing Dock,Ibold Installation"
+ },
+ {
+ "name": "Tsim Binavi",
+ "pos_x": -43.9375,
+ "pos_y": 65.34375,
+ "pos_z": 77.5625,
+ "stations": "Pennington Terminal,Allen Landing"
+ },
+ {
+ "name": "Tsim Binba",
+ "pos_x": 10.0625,
+ "pos_y": -81.125,
+ "pos_z": 1.25,
+ "stations": "Fearn's Inheritance,Kopal Orbital,Chernykh Port,Schouten Plant,Krenkel Vision"
+ },
+ {
+ "name": "Tsim Bind",
+ "pos_x": 100.875,
+ "pos_y": -141.125,
+ "pos_z": 122.96875,
+ "stations": "Safdie Orbital,Baraniecki Vision,Beshore's Progress"
+ },
+ {
+ "name": "Tsimaia",
+ "pos_x": -121.375,
+ "pos_y": -44.59375,
+ "pos_z": -57.875,
+ "stations": "Van Scyoc Station"
+ },
+ {
+ "name": "Tsimaskara",
+ "pos_x": -7.40625,
+ "pos_y": 32.40625,
+ "pos_z": 68.1875,
+ "stations": "Barjavel Port"
+ },
+ {
+ "name": "Tsimshis",
+ "pos_x": 98.78125,
+ "pos_y": 36.15625,
+ "pos_z": -76.90625,
+ "stations": "Aldiss Hub,Baraniecki Colony,Nye Terminal"
+ },
+ {
+ "name": "Tsoghom Na",
+ "pos_x": 108.6875,
+ "pos_y": -100.96875,
+ "pos_z": -34,
+ "stations": "Sucharitkul Survey,Ziegel Mine"
+ },
+ {
+ "name": "Tsoghomal",
+ "pos_x": -115.53125,
+ "pos_y": 60.53125,
+ "pos_z": 35.4375,
+ "stations": "Mach Terminal,Sharma Prospect"
+ },
+ {
+ "name": "Tsogobri",
+ "pos_x": -36.9375,
+ "pos_y": -62.96875,
+ "pos_z": 34.59375,
+ "stations": "Boas Relay,Harrison's Progress"
+ },
+ {
+ "name": "Tsohave",
+ "pos_x": 91.5625,
+ "pos_y": 28.03125,
+ "pos_z": -60.46875,
+ "stations": "Caryanda Enterprise,Sinisalo Ring,Land Terminal,MacDonald Port,Stabenow City,Almagro Station,Sheckley Gateway,Jeschke Ring,Francisco de Eliza Ring"
+ },
+ {
+ "name": "Tsohochosi",
+ "pos_x": 113.03125,
+ "pos_y": -81.46875,
+ "pos_z": 30.84375,
+ "stations": "Collins Prospect,Zenbei Port,Miyasaka Terminal,Blish Vision"
+ },
+ {
+ "name": "Tsohock",
+ "pos_x": 105.125,
+ "pos_y": -40.6875,
+ "pos_z": 25.875,
+ "stations": "Herschel Terminal"
+ },
+ {
+ "name": "Tsohoda",
+ "pos_x": 61.3125,
+ "pos_y": -69.375,
+ "pos_z": 108.21875,
+ "stations": "Vaucouleurs Orbital,Leopold Heckmann Terminal,Dominique Hub,De Lay Port,Filter Hub,Xuesen Base,Ambartsumian Hub"
+ },
+ {
+ "name": "Tsohodiae",
+ "pos_x": -103.75,
+ "pos_y": 30.96875,
+ "pos_z": -1.5625,
+ "stations": "Navigator Platform,Yurchikhin Base,Al-Kashi Prospect,Westerfeld Platform"
+ },
+ {
+ "name": "Tsondama",
+ "pos_x": 57.15625,
+ "pos_y": 77.875,
+ "pos_z": 6.84375,
+ "stations": "Trinh Terminal,Heyerdahl Enterprise,Ciferri Orbital,Leonov Port,Zettel Relay"
+ },
+ {
+ "name": "Tsonensei",
+ "pos_x": -127.90625,
+ "pos_y": -97.4375,
+ "pos_z": -40.03125,
+ "stations": "Tasman Enterprise,Lee Relay,Werber Settlement"
+ },
+ {
+ "name": "Tsongoris",
+ "pos_x": -84.84375,
+ "pos_y": -12.40625,
+ "pos_z": -93.625,
+ "stations": "Meikle Dock,Carpenter Dock,Savitskaya Orbital,Gerst City,Alenquer's Pride,Engle City,Liska Port,Pudwill Gorie Station,Duffy Station,Haignere Forum,Lounge Vision,Bresnik Keep,Mitra Beacon,Citi Vision"
+ },
+ {
+ "name": "Tsu",
+ "pos_x": -8.40625,
+ "pos_y": 99.1875,
+ "pos_z": 22.3125,
+ "stations": "Ziemkiewicz Ring,Reamy Terminal,Doyle Gateway"
+ },
+ {
+ "name": "Tsui",
+ "pos_x": -163.90625,
+ "pos_y": 25.40625,
+ "pos_z": 0.3125,
+ "stations": "Perry Station,Greenleaf Landing,de Balboa Base"
+ },
+ {
+ "name": "Tsuki Yomi",
+ "pos_x": -132.125,
+ "pos_y": 42.53125,
+ "pos_z": 68.21875,
+ "stations": "Chalker Terminal,Stefansson Vision,Cook Orbital"
+ },
+ {
+ "name": "Tswarijem",
+ "pos_x": -133.8125,
+ "pos_y": 102.28125,
+ "pos_z": -21.78125,
+ "stations": "Andreas Works,Pournelle Landing"
+ },
+ {
+ "name": "Tswati",
+ "pos_x": -84,
+ "pos_y": 39.5,
+ "pos_z": -107.28125,
+ "stations": "Dukaj Landing,Robson Point,Lopez de Haro's Pride"
+ },
+ {
+ "name": "TT 58343",
+ "pos_x": -65.1875,
+ "pos_y": -12.84375,
+ "pos_z": -29,
+ "stations": "Jendrassik Port,Stephenson Camp"
+ },
+ {
+ "name": "Tuara",
+ "pos_x": -56.5625,
+ "pos_y": -104.375,
+ "pos_z": 63.28125,
+ "stations": "Pacheco Arsenal,Cesar Janssen Hangar,Searle Refinery"
+ },
+ {
+ "name": "Tuareg",
+ "pos_x": 110.84375,
+ "pos_y": -158.375,
+ "pos_z": -2.3125,
+ "stations": "Albumasar City,Kopff Dock"
+ },
+ {
+ "name": "Tuarezo",
+ "pos_x": 44.59375,
+ "pos_y": -91.90625,
+ "pos_z": 63.125,
+ "stations": "Johnson Platform"
+ },
+ {
+ "name": "Tub Bajo",
+ "pos_x": 107.96875,
+ "pos_y": -4.46875,
+ "pos_z": 45.25,
+ "stations": "Bellamy Arsenal,Beckman Platform,Revin Outpost"
+ },
+ {
+ "name": "Tubalith",
+ "pos_x": 168.5,
+ "pos_y": -175,
+ "pos_z": 57.15625,
+ "stations": "Winthrop Vision,Potocnik Hub,Seki Hub,Sauma Landing,Krupkat Installation"
+ },
+ {
+ "name": "Tucanae Sector AF-A d71",
+ "pos_x": 120.84375,
+ "pos_y": -254.09375,
+ "pos_z": 127.09375,
+ "stations": "Gann Arsenal"
+ },
+ {
+ "name": "Tucanae Sector BQ-Y d113",
+ "pos_x": 52.1875,
+ "pos_y": -145.8125,
+ "pos_z": 186.03125,
+ "stations": "Wylie Point"
+ },
+ {
+ "name": "Tucanae Sector BQ-Y d95",
+ "pos_x": 83.59375,
+ "pos_y": -132.8125,
+ "pos_z": 203.0625,
+ "stations": "Arfstrom Depot"
+ },
+ {
+ "name": "Tucanae Sector CQ-Y d73",
+ "pos_x": 160.125,
+ "pos_y": -156.25,
+ "pos_z": 158.21875,
+ "stations": "Pavlou Prospect,Shepard Landing"
+ },
+ {
+ "name": "Tucanae Sector CQ-Y d86",
+ "pos_x": 109.875,
+ "pos_y": -167.625,
+ "pos_z": 194.9375,
+ "stations": "Bohrmann Landing"
+ },
+ {
+ "name": "Tucanae Sector DQ-Y c11",
+ "pos_x": 131.78125,
+ "pos_y": -234.96875,
+ "pos_z": 98.9375,
+ "stations": "Kohoutek Point"
+ },
+ {
+ "name": "Tucanae Sector DQ-Y c5",
+ "pos_x": 117.34375,
+ "pos_y": -243.8125,
+ "pos_z": 109.65625,
+ "stations": "Phillips Enterprise"
+ },
+ {
+ "name": "Tucanae Sector DQ-Y c6",
+ "pos_x": 100.5,
+ "pos_y": -256.25,
+ "pos_z": 95.625,
+ "stations": "Holub Observatory"
+ },
+ {
+ "name": "Tujia",
+ "pos_x": -113.125,
+ "pos_y": 17.03125,
+ "pos_z": 5.65625,
+ "stations": "Pauling Park,Napier Hub,Shelley Station,Fernao do Po Hub,Walters Orbital,Asire Port,H. G. Wells Terminal,Macedo Holdings"
+ },
+ {
+ "name": "Tujil",
+ "pos_x": 144.9375,
+ "pos_y": -13.21875,
+ "pos_z": 42.34375,
+ "stations": "Hoard Orbital,Bixby Base,Curie Hub,Dirac Terminal,Bounds Works"
+ },
+ {
+ "name": "Tujila",
+ "pos_x": -64.375,
+ "pos_y": -62.875,
+ "pos_z": -153.875,
+ "stations": "Fernao do Po Orbital,Vonnegut Dock,Herbert Keep"
+ },
+ {
+ "name": "Tujina",
+ "pos_x": -47.9375,
+ "pos_y": 78,
+ "pos_z": 134.1875,
+ "stations": "Sinclair Prospect"
+ },
+ {
+ "name": "Tujing",
+ "pos_x": 147.125,
+ "pos_y": -164.78125,
+ "pos_z": 21.125,
+ "stations": "Vaisala Ring,Thiele Gateway,Altshuller Relay,Arago Survey"
+ },
+ {
+ "name": "Tukatjanino",
+ "pos_x": 39.28125,
+ "pos_y": 33.15625,
+ "pos_z": 97.65625,
+ "stations": "Galiano Mines"
+ },
+ {
+ "name": "Tuku",
+ "pos_x": 149.75,
+ "pos_y": -128.75,
+ "pos_z": -52.5625,
+ "stations": "Libeskind Depot"
+ },
+ {
+ "name": "Tukualang",
+ "pos_x": 2.1875,
+ "pos_y": 73,
+ "pos_z": -51.65625,
+ "stations": "Davidson Terminal,Zajdel Station,Ross Legacy"
+ },
+ {
+ "name": "Tukuluma",
+ "pos_x": 102.90625,
+ "pos_y": 123.03125,
+ "pos_z": -38.71875,
+ "stations": "Conti Stop,Low Penal colony"
+ },
+ {
+ "name": "Tukur",
+ "pos_x": 24.875,
+ "pos_y": 46.5625,
+ "pos_z": 89.84375,
+ "stations": "Charnas Installation,Roberts Camp"
+ },
+ {
+ "name": "Tulill",
+ "pos_x": -25.4375,
+ "pos_y": 53.09375,
+ "pos_z": 90.65625,
+ "stations": "Hassanein Dock,Griffith Enterprise,Desargues Ring,Harness Horizons,Hiraga Base"
+ },
+ {
+ "name": "Tulua",
+ "pos_x": 98.15625,
+ "pos_y": -95.46875,
+ "pos_z": 86.4375,
+ "stations": "Chandra Vision,Shaara Vision"
+ },
+ {
+ "name": "Tuluraci",
+ "pos_x": -44.34375,
+ "pos_y": -86.84375,
+ "pos_z": 129.625,
+ "stations": "Fraas Survey,Lorenz Installation,Ockels Landing"
+ },
+ {
+ "name": "Tum",
+ "pos_x": 16.75,
+ "pos_y": -43.71875,
+ "pos_z": -23.03125,
+ "stations": "Elcano Keep,Park Station"
+ },
+ {
+ "name": "Tumbarentii",
+ "pos_x": 29.59375,
+ "pos_y": -118.125,
+ "pos_z": -101.96875,
+ "stations": "Houssay Depot,Nikitin Barracks,Wright Works"
+ },
+ {
+ "name": "Tumuye",
+ "pos_x": 85.90625,
+ "pos_y": 17.59375,
+ "pos_z": -82.28125,
+ "stations": "Siegel Camp"
+ },
+ {
+ "name": "Tumuzgo",
+ "pos_x": 138.78125,
+ "pos_y": -134.6875,
+ "pos_z": 78.9375,
+ "stations": "Hulse Mines,Chadbourne Settlement,Luu Hub,Izumikawa Prison"
+ },
+ {
+ "name": "Tun",
+ "pos_x": -47.96875,
+ "pos_y": 20.96875,
+ "pos_z": -17.53125,
+ "stations": "Tun's Wart"
+ },
+ {
+ "name": "Tungus",
+ "pos_x": -30.09375,
+ "pos_y": 40.1875,
+ "pos_z": 130,
+ "stations": "Blaschke Station,Nachtigal's Inheritance,Bixby Station,Moorcock Enterprise,Maybury Relay"
+ },
+ {
+ "name": "Tunuvii",
+ "pos_x": 126.28125,
+ "pos_y": -15.46875,
+ "pos_z": 64.34375,
+ "stations": "Murdoch Survey,Stephenson Enterprise,Butcher Prison"
+ },
+ {
+ "name": "Tupa",
+ "pos_x": -63.625,
+ "pos_y": -9.96875,
+ "pos_z": 0.0625,
+ "stations": "Leopold Reserve,Montrose Vision"
+ },
+ {
+ "name": "Tupeng Yu",
+ "pos_x": 41.59375,
+ "pos_y": -18.15625,
+ "pos_z": 73.875,
+ "stations": "Robinson Terminal"
+ },
+ {
+ "name": "Turbacobo",
+ "pos_x": -23.53125,
+ "pos_y": -123.625,
+ "pos_z": -10.75,
+ "stations": "Chernykh City"
+ },
+ {
+ "name": "Turbela",
+ "pos_x": -15.34375,
+ "pos_y": -41.78125,
+ "pos_z": 137.03125,
+ "stations": "Rayhan al-Biruni Base"
+ },
+ {
+ "name": "Turberamoan",
+ "pos_x": 104.3125,
+ "pos_y": -1.15625,
+ "pos_z": 62.71875,
+ "stations": "Kondratyev Barracks,Samuda Freeport"
+ },
+ {
+ "name": "Turbo",
+ "pos_x": 47.34375,
+ "pos_y": -52.96875,
+ "pos_z": -121.90625,
+ "stations": "Utley Vision,Kanwar Horizons,Crook Point,Priestley Colony,Pausch Installation"
+ },
+ {
+ "name": "Turboletae",
+ "pos_x": 89.21875,
+ "pos_y": 130.46875,
+ "pos_z": -66.21875,
+ "stations": "Barnes Mine,Kelly Terminal"
+ },
+ {
+ "name": "Turd Wu",
+ "pos_x": -106.125,
+ "pos_y": -0.34375,
+ "pos_z": 72.28125,
+ "stations": "Taylor Point,Reilly City,Bunsen Works"
+ },
+ {
+ "name": "Turd Wura",
+ "pos_x": -77.65625,
+ "pos_y": -29.375,
+ "pos_z": 42.25,
+ "stations": "Hottot Beacon"
+ },
+ {
+ "name": "Turdet",
+ "pos_x": 83.5,
+ "pos_y": -77.46875,
+ "pos_z": -66.1875,
+ "stations": "Robinson's Progress,Meredith Survey,Powell Settlement"
+ },
+ {
+ "name": "Turdetani",
+ "pos_x": 67.09375,
+ "pos_y": 39.65625,
+ "pos_z": 55.3125,
+ "stations": "Rushworth Port,Popov Colony"
+ },
+ {
+ "name": "Turigame",
+ "pos_x": 4.03125,
+ "pos_y": -1.34375,
+ "pos_z": 135.5,
+ "stations": "Artin Escape,Ivanov Station,Galton Port"
+ },
+ {
+ "name": "Turing",
+ "pos_x": 41.9375,
+ "pos_y": 54.375,
+ "pos_z": 124.5625,
+ "stations": "Hart Terminal,Boe Hub,Bulleid Terminal,Killough Installation"
+ },
+ {
+ "name": "Turingga",
+ "pos_x": -1.8125,
+ "pos_y": -154.71875,
+ "pos_z": 105.25,
+ "stations": "Grunsfeld Port"
+ },
+ {
+ "name": "Turir",
+ "pos_x": 29.5,
+ "pos_y": -75.5,
+ "pos_z": 58.1875,
+ "stations": "Brooks Terminal,Lethem Terminal,Cayley Exchange,Isherwood City"
+ },
+ {
+ "name": "Turmakul",
+ "pos_x": -79.1875,
+ "pos_y": 64.25,
+ "pos_z": -33.875,
+ "stations": "O'Brien Horizons,Alpers Works,Stabenow Installation"
+ },
+ {
+ "name": "Turmati",
+ "pos_x": 22.9375,
+ "pos_y": -31.8125,
+ "pos_z": -40.28125,
+ "stations": "Tyurin Horizons,Cochrane Base"
+ },
+ {
+ "name": "Turmatis",
+ "pos_x": -69.34375,
+ "pos_y": 63.84375,
+ "pos_z": -84.0625,
+ "stations": "Crowley Base,Nesvadba Terminal"
+ },
+ {
+ "name": "Turmo",
+ "pos_x": -42.03125,
+ "pos_y": -42.25,
+ "pos_z": 147.75,
+ "stations": "Tokubei Prospect,Vancouver's Inheritance,Anthony de la Roche Holdings"
+ },
+ {
+ "name": "Turna",
+ "pos_x": -18.3125,
+ "pos_y": 58.71875,
+ "pos_z": -67.28125,
+ "stations": "Chwedyk Terminal,Cooper Depot"
+ },
+ {
+ "name": "Turnat",
+ "pos_x": 92.28125,
+ "pos_y": -18.84375,
+ "pos_z": -27.375,
+ "stations": "Lorenz Ring,Conway Terminal,Cenker Orbital,Pierres Holdings,Pangborn Penal colony"
+ },
+ {
+ "name": "Turngin",
+ "pos_x": -114.78125,
+ "pos_y": -34.53125,
+ "pos_z": 66.25,
+ "stations": "Jordan Plant,Herreshoff Colony,Xuanzang's Folly"
+ },
+ {
+ "name": "Turni",
+ "pos_x": 147.125,
+ "pos_y": 35.4375,
+ "pos_z": -3.46875,
+ "stations": "Liska Hub,Murray Orbital,Hugh Enterprise,Gordon Hub"
+ },
+ {
+ "name": "Turnon",
+ "pos_x": -85.75,
+ "pos_y": 41.625,
+ "pos_z": 51.375,
+ "stations": "Hurston Hub"
+ },
+ {
+ "name": "Turo",
+ "pos_x": 155.125,
+ "pos_y": -100.53125,
+ "pos_z": -60.8125,
+ "stations": "Fraknoi Hub"
+ },
+ {
+ "name": "Turomes",
+ "pos_x": 86.25,
+ "pos_y": -122.53125,
+ "pos_z": 101.78125,
+ "stations": "Reinmuth Port,Kuiper Point"
+ },
+ {
+ "name": "Turona",
+ "pos_x": 84.78125,
+ "pos_y": -19.5625,
+ "pos_z": 86.28125,
+ "stations": "McNair Terminal,Bohnhoff Installation,Barnwell Landing"
+ },
+ {
+ "name": "Turong",
+ "pos_x": 111.875,
+ "pos_y": -196.1875,
+ "pos_z": 79.03125,
+ "stations": "Fox Terminal"
+ },
+ {
+ "name": "Turongma",
+ "pos_x": -80.96875,
+ "pos_y": 73.28125,
+ "pos_z": 81.75,
+ "stations": "Stephenson Colony,Perga Dock"
+ },
+ {
+ "name": "Turot",
+ "pos_x": -21.34375,
+ "pos_y": 6.4375,
+ "pos_z": 82.375,
+ "stations": "Hornby Vision,Moon Prospect"
+ },
+ {
+ "name": "Turots",
+ "pos_x": -39.625,
+ "pos_y": 79.125,
+ "pos_z": 104.625,
+ "stations": "Gunn Platform,Napier Refinery"
+ },
+ {
+ "name": "Tutumu",
+ "pos_x": -6.75,
+ "pos_y": 111.1875,
+ "pos_z": -124.21875,
+ "stations": "North Ring,Vogel Penal colony"
+ },
+ {
+ "name": "Tutumuye",
+ "pos_x": 27.40625,
+ "pos_y": -18.96875,
+ "pos_z": 144.9375,
+ "stations": "Franklin Port,Bernoulli Terminal,Rukavishnikov Depot"
+ },
+ {
+ "name": "Tuva",
+ "pos_x": 86.46875,
+ "pos_y": 138.9375,
+ "pos_z": 60.21875,
+ "stations": "Poisson Reach,Alexandria Colony,Dias Enterprise"
+ },
+ {
+ "name": "Tuvan",
+ "pos_x": 57.21875,
+ "pos_y": 29,
+ "pos_z": 115.46875,
+ "stations": "Noriega Survey"
+ },
+ {
+ "name": "Tuvana",
+ "pos_x": 58.03125,
+ "pos_y": -149.5,
+ "pos_z": 144.9375,
+ "stations": "Salam Relay,Mori Depot"
+ },
+ {
+ "name": "Tuvans",
+ "pos_x": -126.46875,
+ "pos_y": -76.65625,
+ "pos_z": 13.0625,
+ "stations": "Coles Relay,Christopher Station"
+ },
+ {
+ "name": "Tvasta",
+ "pos_x": -83.625,
+ "pos_y": -61.78125,
+ "pos_z": 135.15625,
+ "stations": "Serrao Landing,Malzberg Dock,Navigator Landing,Teng Prospect"
+ },
+ {
+ "name": "Tvastyas",
+ "pos_x": -52.875,
+ "pos_y": 128.53125,
+ "pos_z": -14.96875,
+ "stations": "Howard Terminal,Jones' Inheritance,Bates Ring"
+ },
+ {
+ "name": "Tvasu",
+ "pos_x": -123.6875,
+ "pos_y": -52,
+ "pos_z": -17,
+ "stations": "Levinson Horizons,Marques Port,Biggle City,Rosenberg Point"
+ },
+ {
+ "name": "Tvasuki",
+ "pos_x": 41.375,
+ "pos_y": 26.15625,
+ "pos_z": 53.96875,
+ "stations": "Thompson Installation,Pullman Colony"
+ },
+ {
+ "name": "Tvasus",
+ "pos_x": 69.4375,
+ "pos_y": 38.46875,
+ "pos_z": 93.46875,
+ "stations": "Potter Outpost,Heng Horizons"
+ },
+ {
+ "name": "Two Ladies",
+ "pos_x": 19.625,
+ "pos_y": -51.8125,
+ "pos_z": 37.8125,
+ "stations": "Shinn Orbital,Julian Hub,Beekman Platform,Betancourt Horizons"
+ },
+ {
+ "name": "Txamsa",
+ "pos_x": 186.6875,
+ "pos_y": -105.46875,
+ "pos_z": 17.84375,
+ "stations": "Cellarius Colony,Finlay Terminal,i Sola Vision,Bishop Bastion"
+ },
+ {
+ "name": "Txamsem",
+ "pos_x": -2.65625,
+ "pos_y": 99.75,
+ "pos_z": 124.84375,
+ "stations": "Richards Point,Ramsbottom Works"
+ },
+ {
+ "name": "Txamsemi",
+ "pos_x": 158.84375,
+ "pos_y": 56.15625,
+ "pos_z": 4.1875,
+ "stations": "Farrukh Platform,Barth Landing,Waldrop Refinery"
+ },
+ {
+ "name": "TYC 82-953-1",
+ "pos_x": 21.1875,
+ "pos_y": -48.9375,
+ "pos_z": -82.71875,
+ "stations": "Archambault Survey,Still Works,Moore Barracks"
+ },
+ {
+ "name": "Tyche",
+ "pos_x": 25.21875,
+ "pos_y": 43.96875,
+ "pos_z": -28.6875,
+ "stations": "Olivas Survey,Nikolayev's Claim"
+ },
+ {
+ "name": "Tyerisu",
+ "pos_x": 0.53125,
+ "pos_y": -64.84375,
+ "pos_z": 115.84375,
+ "stations": "Rusch Asylum"
+ },
+ {
+ "name": "Tyerremon",
+ "pos_x": 119.21875,
+ "pos_y": -19.40625,
+ "pos_z": -80.03125,
+ "stations": "Barcelo Dock,Barton Penal colony"
+ },
+ {
+ "name": "Tylis",
+ "pos_x": 134.1875,
+ "pos_y": 40.40625,
+ "pos_z": 3.3125,
+ "stations": "Hayden Camp,Forsskal Installation"
+ },
+ {
+ "name": "Tyr",
+ "pos_x": -79.625,
+ "pos_y": 21.25,
+ "pos_z": -25.0625,
+ "stations": "Glashow Dock,Sinclair Base,Fanning Relay"
+ },
+ {
+ "name": "Tyrfing",
+ "pos_x": -57.625,
+ "pos_y": -83.4375,
+ "pos_z": 25.21875,
+ "stations": "Chios Gateway,Cheli City"
+ },
+ {
+ "name": "TZ Arietis",
+ "pos_x": -5.375,
+ "pos_y": -10.59375,
+ "pos_z": -8.5,
+ "stations": "Snyder Enterprise,Puleston Horizons,McDaniel Landing,Godwin Prospect,Santos Depot"
+ },
+ {
+ "name": "Tz'utuls",
+ "pos_x": -57.4375,
+ "pos_y": -89.1875,
+ "pos_z": 23.0625,
+ "stations": "Kanwar Orbital,Gamow Terminal,Anderson Barracks,Onufriyenko Landing"
+ },
+ {
+ "name": "Tz'utumu",
+ "pos_x": -86.21875,
+ "pos_y": -99.5,
+ "pos_z": -75,
+ "stations": "Lundwall Port,Sternberg Depot,Ericsson Bastion"
+ },
+ {
+ "name": "Tzel",
+ "pos_x": -54.40625,
+ "pos_y": -82.65625,
+ "pos_z": 6.625,
+ "stations": "Hennen Port"
+ },
+ {
+ "name": "Tzeltal",
+ "pos_x": -118.5,
+ "pos_y": -81.125,
+ "pos_z": -76.9375,
+ "stations": "Lenthall Hub,Howard Port,Maybury's Inheritance"
+ },
+ {
+ "name": "Tzotz",
+ "pos_x": -134.96875,
+ "pos_y": -6.78125,
+ "pos_z": -16.625,
+ "stations": "Linenger Mines"
+ },
+ {
+ "name": "Tzotzicni",
+ "pos_x": 30.46875,
+ "pos_y": -14.8125,
+ "pos_z": 111.4375,
+ "stations": "Piercy Colony,Haldeman Orbital,Rayhan al-Biruni Depot"
+ },
+ {
+ "name": "Tzotzil",
+ "pos_x": -14.09375,
+ "pos_y": 76.71875,
+ "pos_z": 66.3125,
+ "stations": "Alvares Oasis,Great Prospect,Asaro Silo"
+ },
+ {
+ "name": "Tzu I",
+ "pos_x": -51.28125,
+ "pos_y": -49.09375,
+ "pos_z": 37.71875,
+ "stations": "Howard Enterprise,Farrer Dock"
+ },
+ {
+ "name": "Tzu Kun",
+ "pos_x": 26.21875,
+ "pos_y": -53.46875,
+ "pos_z": -143.96875,
+ "stations": "Klein Settlement"
+ },
+ {
+ "name": "u Carinae",
+ "pos_x": 90.0625,
+ "pos_y": 0.78125,
+ "pos_z": 30.1875,
+ "stations": "Elion Vision,Carpenter Gateway,Glidden Ring,Kubasov Terminal,Steinmuller's Folly,Herbert Landing,Molina Orbital,Rennie City"
+ },
+ {
+ "name": "Uadjala",
+ "pos_x": 36.375,
+ "pos_y": -139.40625,
+ "pos_z": 100.5625,
+ "stations": "Frimout Dock"
+ },
+ {
+ "name": "Uadjamat",
+ "pos_x": 40.34375,
+ "pos_y": -80.5,
+ "pos_z": 102.40625,
+ "stations": "Hafner Enterprise,Kelly Refinery,Loewy Orbital"
+ },
+ {
+ "name": "Uadjaramin",
+ "pos_x": -112.15625,
+ "pos_y": -71.25,
+ "pos_z": 50.03125,
+ "stations": "Samokutyayev Terminal,Alas Retreat"
+ },
+ {
+ "name": "Uadjarii",
+ "pos_x": -105.96875,
+ "pos_y": -97.46875,
+ "pos_z": 25.34375,
+ "stations": "Asimov Platform,O'Donnell Colony"
+ },
+ {
+ "name": "Uadjiru",
+ "pos_x": 130.40625,
+ "pos_y": -72.3125,
+ "pos_z": 41.46875,
+ "stations": "Curtis Gateway,Rawat Survey,Carnera Ring,Anthony de la Roche Prospect"
+ },
+ {
+ "name": "Ualaallung",
+ "pos_x": 68.78125,
+ "pos_y": -173.96875,
+ "pos_z": 144.65625,
+ "stations": "Ryan Landing,Tusi Station,Marsden Base"
+ },
+ {
+ "name": "Ualak",
+ "pos_x": 38.90625,
+ "pos_y": -104.40625,
+ "pos_z": -49.96875,
+ "stations": "Hawke Dock"
+ },
+ {
+ "name": "Ualam",
+ "pos_x": 20.5,
+ "pos_y": -97.6875,
+ "pos_z": -139.90625,
+ "stations": "Franklin Depot"
+ },
+ {
+ "name": "Ualama",
+ "pos_x": 73.4375,
+ "pos_y": -117.0625,
+ "pos_z": 172.875,
+ "stations": "Brouwer Port"
+ },
+ {
+ "name": "Ualame",
+ "pos_x": -53.375,
+ "pos_y": 15.84375,
+ "pos_z": 75.75,
+ "stations": "Lessing Hangar"
+ },
+ {
+ "name": "Ualana",
+ "pos_x": 116.84375,
+ "pos_y": 18.4375,
+ "pos_z": -91.84375,
+ "stations": "Moran Platform,McAllaster Colony"
+ },
+ {
+ "name": "Ualangur",
+ "pos_x": -74.46875,
+ "pos_y": -30.3125,
+ "pos_z": 38.53125,
+ "stations": "Harness Installation,Atkov Enterprise,Baydukov Point"
+ },
+ {
+ "name": "Ualapalor",
+ "pos_x": -20.375,
+ "pos_y": -10.59375,
+ "pos_z": 65.78125,
+ "stations": "Leichhardt City,Preuss Station,Rochon Ring,Nesvadba Dock,Anderson Terminal,Wagner City"
+ },
+ {
+ "name": "Ualar",
+ "pos_x": 143.59375,
+ "pos_y": -143.78125,
+ "pos_z": 20.4375,
+ "stations": "Rubin Ring,Unsold Terminal,Meech Terminal,Hoyle Palace,van Rhijn Prospect,Gordon Relay"
+ },
+ {
+ "name": "Ualarni",
+ "pos_x": 214.5625,
+ "pos_y": -174.34375,
+ "pos_z": 22.25,
+ "stations": "Nadiradze Horizons,d'Arrest Landing,Kranz Dock,Tousey Holdings,Wilder Depot"
+ },
+ {
+ "name": "Ualaroges",
+ "pos_x": -39.40625,
+ "pos_y": -69.28125,
+ "pos_z": 137.96875,
+ "stations": "Alpers Base,Gooch Works"
+ },
+ {
+ "name": "Uayeb",
+ "pos_x": 65.03125,
+ "pos_y": 89.25,
+ "pos_z": 105.875,
+ "stations": "Goldstein Terminal,Gilliland's Claim,Crown Depot"
+ },
+ {
+ "name": "Uayeb Xoc",
+ "pos_x": 163.15625,
+ "pos_y": 26.375,
+ "pos_z": 47.78125,
+ "stations": "Reamy Port"
+ },
+ {
+ "name": "Uayehi",
+ "pos_x": -45.5625,
+ "pos_y": 95.03125,
+ "pos_z": -22,
+ "stations": "Kennedy Terminal,Auer Point,Clauss Orbital"
+ },
+ {
+ "name": "Uba",
+ "pos_x": 182.125,
+ "pos_y": -146.3125,
+ "pos_z": -3.15625,
+ "stations": "Hoyle Enterprise"
+ },
+ {
+ "name": "Ubassi",
+ "pos_x": -104.90625,
+ "pos_y": 72.21875,
+ "pos_z": 77.75,
+ "stations": "Harness Base,Bobko Orbital,Bloomfield Platform"
+ },
+ {
+ "name": "Ubastet",
+ "pos_x": 126.8125,
+ "pos_y": 89.1875,
+ "pos_z": -103.8125,
+ "stations": "Abernathy Station,Conti Holdings,Abbott Landing"
+ },
+ {
+ "name": "Uberadji",
+ "pos_x": 1.15625,
+ "pos_y": -211.40625,
+ "pos_z": 23.53125,
+ "stations": "Fowler Survey,Makarov Relay,Phillips Vision,Hyecho Keep"
+ },
+ {
+ "name": "Ubere",
+ "pos_x": -100.40625,
+ "pos_y": 28.125,
+ "pos_z": -28.75,
+ "stations": "Spassky Vision,Bradley Dock,Pryor Gateway,Nansen Hub"
+ },
+ {
+ "name": "UBV 15076",
+ "pos_x": -25.78125,
+ "pos_y": 14.03125,
+ "pos_z": 104.8125,
+ "stations": "Carey Enterprise,Covey Hub,Georg Bothe Hub,Tiedemann Relay,Eckford Horizons"
+ },
+ {
+ "name": "UBV 8670",
+ "pos_x": 78.15625,
+ "pos_y": -8.6875,
+ "pos_z": 4.78125,
+ "stations": "Fettman Enterprise,Schlegel Terminal,Moseley Hub,Hurley Dock,Eschbach Refinery,Mallett Terminal,Midgley Hub,Greenland Silo"
+ },
+ {
+ "name": "Uchagan",
+ "pos_x": -81.84375,
+ "pos_y": -7.28125,
+ "pos_z": -2.9375,
+ "stations": "Gagarin Camp,Watt Base"
+ },
+ {
+ "name": "Uchaluroja",
+ "pos_x": -10.09375,
+ "pos_y": 0.3125,
+ "pos_z": -56.875,
+ "stations": "Rozhdestvensky Station,Farmer Terminal,Zudov Dock,Crown Market,Marshall Hub,Willis Dock,Hoften Enterprise,Harrison Orbital,Runco Ring,Illy Installation,Coulomb Works,Disch Keep,Leiber Installation,Cardano Holdings,Clark Prospect"
+ },
+ {
+ "name": "Uchang",
+ "pos_x": 147.21875,
+ "pos_y": -13.34375,
+ "pos_z": 63.71875,
+ "stations": "Fisher Ring"
+ },
+ {
+ "name": "Udegobo",
+ "pos_x": 119.96875,
+ "pos_y": -10.3125,
+ "pos_z": -80.375,
+ "stations": "Roentgen City,Yaping City,Bushkov Camp,Kozeyev Survey"
+ },
+ {
+ "name": "Udegoci",
+ "pos_x": -70,
+ "pos_y": 51.71875,
+ "pos_z": -54.5,
+ "stations": "Kroehl Orbital,Banks Port,Nikitin Landing,Russo Dock,van Vogt Landing"
+ },
+ {
+ "name": "Udihikoja",
+ "pos_x": 12.40625,
+ "pos_y": -79.625,
+ "pos_z": 26.96875,
+ "stations": "Ivanishin Enterprise,Sinclair Landing,Bamford Station"
+ },
+ {
+ "name": "Uellaturt",
+ "pos_x": 204.34375,
+ "pos_y": -140.15625,
+ "pos_z": 16.5625,
+ "stations": "Galle's Claim,Clough's Inheritance"
+ },
+ {
+ "name": "Uelliano",
+ "pos_x": -46.59375,
+ "pos_y": -166.46875,
+ "pos_z": -40.875,
+ "stations": "Tolstoi Depot,Vaisala Station,Kerimov Terminal"
+ },
+ {
+ "name": "Uelliodhino",
+ "pos_x": -60.78125,
+ "pos_y": -74.84375,
+ "pos_z": -22.875,
+ "stations": "Haise Base,Fujimori Base,Banks Penitentiary"
+ },
+ {
+ "name": "Uelloskima",
+ "pos_x": -76.25,
+ "pos_y": -0.46875,
+ "pos_z": 16.875,
+ "stations": "Hedley City,Butz Market,Comer's Progress,Malocello Depot,Macdonald Hub,Davidson's Progress,Dias Camp,Gell-Mann Oasis,Baker's Progress"
+ },
+ {
+ "name": "Uenne",
+ "pos_x": 85.4375,
+ "pos_y": -41.46875,
+ "pos_z": -50.25,
+ "stations": "Gorbatko Port,Davy Landing,Serebrov Enterprise"
+ },
+ {
+ "name": "UGP 120",
+ "pos_x": 75.59375,
+ "pos_y": -51.5,
+ "pos_z": -62.4375,
+ "stations": "Fontenay Port,Stuart Orbital,Nixon City,Matthews Gateway,Hiraga Ring,McKee Horizons,Amundsen City,Bogdanov Station,Fearn Orbital,Kirk Arsenal,Zelazny Gateway"
+ },
+ {
+ "name": "UGP 145",
+ "pos_x": 74.34375,
+ "pos_y": -32.78125,
+ "pos_z": -6.625,
+ "stations": "Barnwell Station,Wollheim Ring,Anvil Terminal,Shiras Orbital,Rescue Ship - Barnwell Station"
+ },
+ {
+ "name": "UGP 184",
+ "pos_x": 106.21875,
+ "pos_y": -32.84375,
+ "pos_z": 24.28125,
+ "stations": "Bohrmann Terminal,Beshore Station,Hansteen Hub,Lambert Arsenal"
+ },
+ {
+ "name": "UGP 485",
+ "pos_x": -40.59375,
+ "pos_y": -49.25,
+ "pos_z": 100.9375,
+ "stations": "Rawat Dock,Grunsfeld Observatory,Heck Lab,Kurland Beacon,Jemison Barracks"
+ },
+ {
+ "name": "UGP 619",
+ "pos_x": -6.65625,
+ "pos_y": -80.8125,
+ "pos_z": 17.53125,
+ "stations": "Gromov City,Barreiros Orbital,Dias Port,Tarentum Hub,Gentle Port"
+ },
+ {
+ "name": "UGP 64",
+ "pos_x": 13.3125,
+ "pos_y": -76.25,
+ "pos_z": -83.9375,
+ "stations": "Glashow Enterprise"
+ },
+ {
+ "name": "UGP 92",
+ "pos_x": 34.78125,
+ "pos_y": -44.46875,
+ "pos_z": -62.8125,
+ "stations": "Deluc Platform,Elcano Penitentiary"
+ },
+ {
+ "name": "Ugras",
+ "pos_x": 148.9375,
+ "pos_y": -23.0625,
+ "pos_z": -12.90625,
+ "stations": "Froud Landing,Zubrin Refinery,Bounds Settlement"
+ },
+ {
+ "name": "Ugraseno",
+ "pos_x": 165.78125,
+ "pos_y": -78.65625,
+ "pos_z": 61.625,
+ "stations": "Giacobini Dock,Gabriel Terminal,Canty Orbital,Mikoyan Works,Wild Terminal"
+ },
+ {
+ "name": "Ugrashtrim",
+ "pos_x": -10.6875,
+ "pos_y": -83.15625,
+ "pos_z": 18.8125,
+ "stations": "Priestley Dock,Northrop Orbital,Bobko Orbital,Shriver Gateway,Ising Terminal,Aleksandrov Station"
+ },
+ {
+ "name": "Ugrasin",
+ "pos_x": -106.71875,
+ "pos_y": 88.625,
+ "pos_z": 11.28125,
+ "stations": "Kuo Settlement"
+ },
+ {
+ "name": "Ugrasmat",
+ "pos_x": -67.5625,
+ "pos_y": -15.71875,
+ "pos_z": -111.53125,
+ "stations": "Dobrovolski Gateway,Babcock Dock,Ellis Dock,Johnson Terminal"
+ },
+ {
+ "name": "Ugraswar",
+ "pos_x": 57.6875,
+ "pos_y": -21.875,
+ "pos_z": 92.625,
+ "stations": "Hermaszewski Ring,Garratt Port,Carr Station,Roberts Terminal,Berliner Port,Zajdel Enterprise,Boucher Vista"
+ },
+ {
+ "name": "Ugraswarka",
+ "pos_x": 55.84375,
+ "pos_y": -126.53125,
+ "pos_z": -94.71875,
+ "stations": "Baille City,Battani Colony,Gustav Sporer City"
+ },
+ {
+ "name": "Ugric",
+ "pos_x": -13.75,
+ "pos_y": -15.21875,
+ "pos_z": -125.3125,
+ "stations": "Sanger Platform,Finney Penal colony"
+ },
+ {
+ "name": "Ugricassur",
+ "pos_x": 150.21875,
+ "pos_y": -189.90625,
+ "pos_z": 60.15625,
+ "stations": "Gehry's Claim"
+ },
+ {
+ "name": "Ugrici",
+ "pos_x": 38.5,
+ "pos_y": -178.375,
+ "pos_z": 60.125,
+ "stations": "Firsoff Dock,Shaw Gateway,Auer Survey,Lundwall Beacon,Rosseland Colony"
+ },
+ {
+ "name": "Ugricopa",
+ "pos_x": 104.375,
+ "pos_y": 21.9375,
+ "pos_z": 81.15625,
+ "stations": "Gunn Refinery,Buchli Enterprise,Viktorenko Port,Chilton Station"
+ },
+ {
+ "name": "Ugrivaci",
+ "pos_x": -76.15625,
+ "pos_y": -66.5,
+ "pos_z": -5.75,
+ "stations": "Stasheff's Folly,Resnik Vision,Adamson Landing"
+ },
+ {
+ "name": "Ugrivirii",
+ "pos_x": 132.5,
+ "pos_y": -99.0625,
+ "pos_z": -3.28125,
+ "stations": "Jefferies Port,Blaauw Depot,Belyavsky Survey"
+ },
+ {
+ "name": "Uhlan",
+ "pos_x": 86.125,
+ "pos_y": -31.5625,
+ "pos_z": -11.6875,
+ "stations": "Pontes Colony"
+ },
+ {
+ "name": "Uhlang",
+ "pos_x": 113.3125,
+ "pos_y": -132,
+ "pos_z": 44.6875,
+ "stations": "Murakami Port,Fraknoi Installation,Safdie Ring,Roed Odegaard Beacon,Nadiradze Relay"
+ },
+ {
+ "name": "Uhlanqui",
+ "pos_x": -65.65625,
+ "pos_y": -101.65625,
+ "pos_z": 63.90625,
+ "stations": "Hennen Port,Parise Hub,Zoline City,Edwards Port,Meikle Hub,Appel Dock,Vaucanson Orbital,Bouch Station,Linnehan Observatory"
+ },
+ {
+ "name": "Uibumisin",
+ "pos_x": -52.25,
+ "pos_y": -90.71875,
+ "pos_z": 88.78125,
+ "stations": "Hubble Hub,Thuot Gateway,Szilard Gateway"
+ },
+ {
+ "name": "Uiburnacomi",
+ "pos_x": 68.40625,
+ "pos_y": -130.71875,
+ "pos_z": 113.8125,
+ "stations": "Naubakht Outpost,Takahashi Beacon"
+ },
+ {
+ "name": "Uiburni",
+ "pos_x": -74.9375,
+ "pos_y": -133.375,
+ "pos_z": -1.1875,
+ "stations": "Brandenstein Vision,Popovich Horizons,Bosch Port,Tudela Base"
+ },
+ {
+ "name": "Uiburoju",
+ "pos_x": -78.25,
+ "pos_y": 27.40625,
+ "pos_z": 122.78125,
+ "stations": "Komarov Port,Stefanyshyn-Piper Colony"
+ },
+ {
+ "name": "Uibus",
+ "pos_x": 137.9375,
+ "pos_y": -75.4375,
+ "pos_z": -39.46875,
+ "stations": "Baillaud Gateway,Denning Gateway,Laurent Keep,Clebsch Vision"
+ },
+ {
+ "name": "Uibuth",
+ "pos_x": 140.8125,
+ "pos_y": -192.96875,
+ "pos_z": 131.1875,
+ "stations": "d'Arrest Station,Albategnius Landing,Fleming Installation,J. G. Ballard Installation"
+ },
+ {
+ "name": "Uke Mebes",
+ "pos_x": 84.53125,
+ "pos_y": -11.5,
+ "pos_z": -131.25,
+ "stations": "Lichtenberg Settlement"
+ },
+ {
+ "name": "Uke Ngura",
+ "pos_x": -109.96875,
+ "pos_y": 24.0625,
+ "pos_z": 101.25,
+ "stations": "Wrangell Refinery,Szebehely Dock,Fuglesang Landing"
+ },
+ {
+ "name": "Ulangin",
+ "pos_x": -47.25,
+ "pos_y": -17.875,
+ "pos_z": -118.65625,
+ "stations": "Wiley Base"
+ },
+ {
+ "name": "Ulanquiates",
+ "pos_x": -42.125,
+ "pos_y": 29.4375,
+ "pos_z": 153.21875,
+ "stations": "Waldrop Dock,Drake Holdings,Stevin Beacon"
+ },
+ {
+ "name": "Ulche",
+ "pos_x": 146.875,
+ "pos_y": 2.375,
+ "pos_z": -106.9375,
+ "stations": "Cowper Settlement,Legendre Point,Okorafor Base"
+ },
+ {
+ "name": "Ulchs",
+ "pos_x": 46.25,
+ "pos_y": 37.8125,
+ "pos_z": -0.8125,
+ "stations": "Scully-Power Dock,Bridges Hangar,Lloyd Point"
+ },
+ {
+ "name": "Ulicunabopa",
+ "pos_x": 100.53125,
+ "pos_y": 93.90625,
+ "pos_z": 106,
+ "stations": "Shelley Ring,Bulychev Dock,Grijalva Park,McCaffrey Survey"
+ },
+ {
+ "name": "Ulla",
+ "pos_x": 20.0625,
+ "pos_y": -15.8125,
+ "pos_z": -171.65625,
+ "stations": "Janszoon Horizons,Norman Works"
+ },
+ {
+ "name": "Ulladan",
+ "pos_x": -14.34375,
+ "pos_y": -195.9375,
+ "pos_z": 37.09375,
+ "stations": "Chernykh Platform,Burke Escape"
+ },
+ {
+ "name": "Ullangwa",
+ "pos_x": 69.84375,
+ "pos_y": -199.375,
+ "pos_z": 32.03125,
+ "stations": "Bouvard Dock,Gurevich Settlement,Rawat Camp"
+ },
+ {
+ "name": "Ulledones",
+ "pos_x": 169.21875,
+ "pos_y": -67.375,
+ "pos_z": 29.28125,
+ "stations": "Kobayashi Base,Dominique Mines,Abnett Relay"
+ },
+ {
+ "name": "Ullemi",
+ "pos_x": 83.46875,
+ "pos_y": 102.375,
+ "pos_z": -2.53125,
+ "stations": "Kubasov Enterprise,Bennett Enterprise,Aleksandrov Port,Burkin Base,Rosenberg Gateway"
+ },
+ {
+ "name": "Ullernobo",
+ "pos_x": -134.59375,
+ "pos_y": 105.0625,
+ "pos_z": -34.65625,
+ "stations": "Key Colony,Evans Freeport,Menzies Base"
+ },
+ {
+ "name": "Ullese",
+ "pos_x": 35.75,
+ "pos_y": -73.78125,
+ "pos_z": 38.96875,
+ "stations": "Roche Works"
+ },
+ {
+ "name": "Ulures",
+ "pos_x": -79.15625,
+ "pos_y": 83.75,
+ "pos_z": -20.4375,
+ "stations": "Hertz Platform,Yang Stop"
+ },
+ {
+ "name": "Uluri",
+ "pos_x": -63.875,
+ "pos_y": 17.28125,
+ "pos_z": 10.15625,
+ "stations": "Parker Beacon,Alvares Penal colony,McDivitt Settlement"
+ },
+ {
+ "name": "Umaiali",
+ "pos_x": 129.90625,
+ "pos_y": 55.40625,
+ "pos_z": 107.15625,
+ "stations": "Leiber Prospect"
+ },
+ {
+ "name": "Umair",
+ "pos_x": -50,
+ "pos_y": -127,
+ "pos_z": 82.21875,
+ "stations": "Schmidt Stop,Hunt's Inheritance"
+ },
+ {
+ "name": "Umairitii",
+ "pos_x": 150.15625,
+ "pos_y": -168.375,
+ "pos_z": -62.25,
+ "stations": "Babakin Landing,Bretnor Bastion"
+ },
+ {
+ "name": "Umait",
+ "pos_x": -100.625,
+ "pos_y": 81.21875,
+ "pos_z": -14.15625,
+ "stations": "Warner Beacon,Strzelecki Port,Clarke Plant"
+ },
+ {
+ "name": "Umaitis",
+ "pos_x": -70.21875,
+ "pos_y": 19.78125,
+ "pos_z": 110.25,
+ "stations": "Stott Station,Drebbel Palace,Stokes Keep"
+ },
+ {
+ "name": "Umaspi",
+ "pos_x": 18.6875,
+ "pos_y": -72.71875,
+ "pos_z": -14.1875,
+ "stations": "Gardner Orbital"
+ },
+ {
+ "name": "Umastae",
+ "pos_x": 137.84375,
+ "pos_y": 40.125,
+ "pos_z": -130.53125,
+ "stations": "Isherwood Dock,Robinson City,Bradbury Enterprise,Stokes Installation,Vasilyev Relay"
+ },
+ {
+ "name": "Umat",
+ "pos_x": 64.46875,
+ "pos_y": -7.375,
+ "pos_z": -133.75,
+ "stations": "Feoktistov Point,Darwin Camp,Kavandi Penal colony"
+ },
+ {
+ "name": "Umathudti",
+ "pos_x": -41.78125,
+ "pos_y": -81.3125,
+ "pos_z": -48.21875,
+ "stations": "Wallace Station"
+ },
+ {
+ "name": "Umatilla",
+ "pos_x": 117.9375,
+ "pos_y": -183.6875,
+ "pos_z": -24.4375,
+ "stations": "Pollas Relay,Kresak Dock"
+ },
+ {
+ "name": "Umbila",
+ "pos_x": -44.3125,
+ "pos_y": -69.4375,
+ "pos_z": -111.0625,
+ "stations": "Tsibliyev Terminal,King Barracks"
+ },
+ {
+ "name": "Umbiri",
+ "pos_x": 166.8125,
+ "pos_y": 30.625,
+ "pos_z": -27.4375,
+ "stations": "Ahmed Enterprise,Wilkes Depot,Campbell Dock"
+ },
+ {
+ "name": "Umbriga",
+ "pos_x": 92.125,
+ "pos_y": 31.6875,
+ "pos_z": -130.125,
+ "stations": "Miletus Mine,Baker Observatory"
+ },
+ {
+ "name": "Umbrigaens",
+ "pos_x": 69.0625,
+ "pos_y": -150.0625,
+ "pos_z": 8.46875,
+ "stations": "Pannekoek Port,Payne-Gaposchkin Station,Kotelnikov Base"
+ },
+ {
+ "name": "Umbrigua",
+ "pos_x": 50.5625,
+ "pos_y": 0.78125,
+ "pos_z": -8.03125,
+ "stations": "Drzewiecki Station,Tokubei Station,Kuo Arsenal,Ballard Orbital"
+ },
+ {
+ "name": "Umbroges",
+ "pos_x": -33.09375,
+ "pos_y": -88.875,
+ "pos_z": -100.28125,
+ "stations": "Jakes' Inheritance,Wylie Settlement"
+ },
+ {
+ "name": "Umbrogo",
+ "pos_x": -3.46875,
+ "pos_y": 79.8125,
+ "pos_z": 59.03125,
+ "stations": "Salk Installation,Arthur Settlement,Bokeili Landing"
+ },
+ {
+ "name": "Umed",
+ "pos_x": -83.6875,
+ "pos_y": -59.28125,
+ "pos_z": 60.28125,
+ "stations": "Stafford Terminal,Nespoli Hub,Cayley Hub,Locke City"
+ },
+ {
+ "name": "Umedet",
+ "pos_x": 17.46875,
+ "pos_y": -109.125,
+ "pos_z": 157.03125,
+ "stations": "Leuschner Vision,Hague Platform,Rosenberger's Progress"
+ },
+ {
+ "name": "Umisii",
+ "pos_x": -58.96875,
+ "pos_y": 57.03125,
+ "pos_z": 125.09375,
+ "stations": "Bogdanov Vision,Humphreys Terminal,Webb Depot,George Laboratory"
+ },
+ {
+ "name": "Umistan",
+ "pos_x": -106.09375,
+ "pos_y": -11.1875,
+ "pos_z": -43.53125,
+ "stations": "Hilmers Installation,Virts Colony,Brin Landing"
+ },
+ {
+ "name": "Umpilakap",
+ "pos_x": -48.28125,
+ "pos_y": -27.875,
+ "pos_z": -17.8125,
+ "stations": "Bushnell Refinery"
+ },
+ {
+ "name": "Umutei",
+ "pos_x": 10.875,
+ "pos_y": 148.40625,
+ "pos_z": 15.90625,
+ "stations": "Wedge Outpost,Parmitano Platform,Steele Base"
+ },
+ {
+ "name": "Umvelis",
+ "pos_x": 111.6875,
+ "pos_y": 84.84375,
+ "pos_z": 65.375,
+ "stations": "Svavarsson Arena"
+ },
+ {
+ "name": "Umvelli",
+ "pos_x": 141.21875,
+ "pos_y": -210.34375,
+ "pos_z": 47.40625,
+ "stations": "Bingzhen Vision,Alexander Depot"
+ },
+ {
+ "name": "Un Nagait",
+ "pos_x": 28.53125,
+ "pos_y": -173.5,
+ "pos_z": 38.71875,
+ "stations": "Mattei Mines,Piano Plant"
+ },
+ {
+ "name": "Un Narraya",
+ "pos_x": 57.5625,
+ "pos_y": -13.75,
+ "pos_z": 4,
+ "stations": "Walker Horizons,Ricci Arsenal,Guest Settlement"
+ },
+ {
+ "name": "Un Nefer",
+ "pos_x": -3.65625,
+ "pos_y": -143.0625,
+ "pos_z": 57.90625,
+ "stations": "Johri Relay"
+ },
+ {
+ "name": "Un No Myoin",
+ "pos_x": 78.1875,
+ "pos_y": -95.28125,
+ "pos_z": -60.875,
+ "stations": "Darwin Point,Searfoss Refinery"
+ },
+ {
+ "name": "Una",
+ "pos_x": -11.09375,
+ "pos_y": 41.09375,
+ "pos_z": 90.53125,
+ "stations": "Felice Hub,Hoard Orbital,Prunariu Orbital,Godwin Port,Darlton Base"
+ },
+ {
+ "name": "Uncegin",
+ "pos_x": 64.8125,
+ "pos_y": 12.40625,
+ "pos_z": 126.28125,
+ "stations": "Filipchenko Stop,Vinge Enterprise"
+ },
+ {
+ "name": "Uncenian",
+ "pos_x": -88.6875,
+ "pos_y": -97.65625,
+ "pos_z": 56.5625,
+ "stations": "Fawcett Terminal,Betancourt Horizons,Garcia Base"
+ },
+ {
+ "name": "Uncenii",
+ "pos_x": -91.78125,
+ "pos_y": 171.8125,
+ "pos_z": -48.90625,
+ "stations": "Coppel Base"
+ },
+ {
+ "name": "Undadja",
+ "pos_x": -108.6875,
+ "pos_y": 24.65625,
+ "pos_z": -20.78125,
+ "stations": "McArthur Hangar,Rutherford Depot"
+ },
+ {
+ "name": "Undalibaluf",
+ "pos_x": -178.6875,
+ "pos_y": 19.4375,
+ "pos_z": -93.40625,
+ "stations": "Beatty Landing,Carpini Relay,Huxley's Inheritance"
+ },
+ {
+ "name": "Undia",
+ "pos_x": -64.25,
+ "pos_y": -182.5,
+ "pos_z": -3.40625,
+ "stations": "Burns Port,Fowler Enterprise"
+ },
+ {
+ "name": "Undin",
+ "pos_x": -88.28125,
+ "pos_y": -64.59375,
+ "pos_z": -79.125,
+ "stations": "Budarin Ring"
+ },
+ {
+ "name": "Undines",
+ "pos_x": -26.90625,
+ "pos_y": -71.21875,
+ "pos_z": 56.09375,
+ "stations": "Cayley Freeport,Shepherd Legacy,Sabine Vista"
+ },
+ {
+ "name": "Undini",
+ "pos_x": -35.3125,
+ "pos_y": 151.65625,
+ "pos_z": 18.09375,
+ "stations": "Cormack's Claim"
+ },
+ {
+ "name": "Unegak Maku",
+ "pos_x": 109.59375,
+ "pos_y": 12.125,
+ "pos_z": 41.71875,
+ "stations": "Tyurin Prospect,Porsche Dock,Lovelace Station,Macarthur Orbital"
+ },
+ {
+ "name": "Uneleni",
+ "pos_x": 116.21875,
+ "pos_y": -196.46875,
+ "pos_z": 114.3125,
+ "stations": "Townes Dock,Biesbroeck Port,Berkey's Progress,Heiles Station"
+ },
+ {
+ "name": "Unelenses",
+ "pos_x": 79.53125,
+ "pos_y": 12.6875,
+ "pos_z": -96.65625,
+ "stations": "Citi Dock,Hawkes Refinery,O'Leary Enterprise"
+ },
+ {
+ "name": "Unelians",
+ "pos_x": 61.9375,
+ "pos_y": -31.25,
+ "pos_z": 139.28125,
+ "stations": "Lonchakov Orbital,Reisman Colony,Hoshide Enterprise"
+ },
+ {
+ "name": "Uneligni",
+ "pos_x": 92.65625,
+ "pos_y": 48.78125,
+ "pos_z": -168.90625,
+ "stations": "Scheerbart Prospect"
+ },
+ {
+ "name": "Unelinte",
+ "pos_x": 99.75,
+ "pos_y": 52.65625,
+ "pos_z": 72.3125,
+ "stations": "Paulmier de Gonneville Station,Cabot City,Moore Dock,Manning Forum,Brooks Landing"
+ },
+ {
+ "name": "Union",
+ "pos_x": -9540.71875,
+ "pos_y": -882.9375,
+ "pos_z": 19810.09375,
+ "stations": "Rebolo Port"
+ },
+ {
+ "name": "Unjadi",
+ "pos_x": 141.71875,
+ "pos_y": -96.40625,
+ "pos_z": 72.5625,
+ "stations": "Wilhelm Klinkerfues Survey"
+ },
+ {
+ "name": "Unjangen",
+ "pos_x": -86.59375,
+ "pos_y": 59.53125,
+ "pos_z": -137.03125,
+ "stations": "Rochon Enterprise,Kelleam Dock,Narita Installation,Geston Survey,Moskowitz Station"
+ },
+ {
+ "name": "Unktety",
+ "pos_x": 118.15625,
+ "pos_y": -150.71875,
+ "pos_z": -82.0625,
+ "stations": "Grigorovich Orbital,Barbuy Vision,Everest Settlement"
+ },
+ {
+ "name": "Unkto",
+ "pos_x": -139.9375,
+ "pos_y": -55.5625,
+ "pos_z": 59.75,
+ "stations": "Glidden Dock,Huygens' Inheritance,Lamarr Dock"
+ },
+ {
+ "name": "Unktock",
+ "pos_x": -41.34375,
+ "pos_y": -14.59375,
+ "pos_z": -68.625,
+ "stations": "Baird Station"
+ },
+ {
+ "name": "Unktomi",
+ "pos_x": -78.625,
+ "pos_y": -21.8125,
+ "pos_z": -31.34375,
+ "stations": "Blish Refinery,Solovyov Dock,Stott Settlement"
+ },
+ {
+ "name": "Unktondjin",
+ "pos_x": -60,
+ "pos_y": -50.5625,
+ "pos_z": 115.09375,
+ "stations": "Bobko Dock"
+ },
+ {
+ "name": "Unkuan Di",
+ "pos_x": -88.875,
+ "pos_y": 91.8125,
+ "pos_z": -58.5,
+ "stations": "Bounds Station,Hawke Hub"
+ },
+ {
+ "name": "Unkuar",
+ "pos_x": -14.15625,
+ "pos_y": 53.96875,
+ "pos_z": 56.28125,
+ "stations": "Flynn Dock,Chalker Installation"
+ },
+ {
+ "name": "Unkulcay",
+ "pos_x": 30.875,
+ "pos_y": -56.84375,
+ "pos_z": -103.46875,
+ "stations": "Eisenstein Dock,Watts Dock,Kozin Platform,Zettel Colony"
+ },
+ {
+ "name": "Unkunder",
+ "pos_x": 119,
+ "pos_y": -53.84375,
+ "pos_z": 84.96875,
+ "stations": "Penzias Settlement,Arrhenius Station,Vercors Point,Fowler Survey"
+ },
+ {
+ "name": "Untuathit",
+ "pos_x": 37.71875,
+ "pos_y": 97.125,
+ "pos_z": 18.3125,
+ "stations": "Jones Plant,Zalyotin Observatory"
+ },
+ {
+ "name": "Untuatucate",
+ "pos_x": 1.1875,
+ "pos_y": -163.6875,
+ "pos_z": 74.65625,
+ "stations": "Cuffey Landing,Linge Vision,Dugan Vision"
+ },
+ {
+ "name": "Untun",
+ "pos_x": 103.5,
+ "pos_y": -97.25,
+ "pos_z": 57.4375,
+ "stations": "Eggleton Stop"
+ },
+ {
+ "name": "Untup",
+ "pos_x": 5.125,
+ "pos_y": -119.78125,
+ "pos_z": 32.21875,
+ "stations": "Penrose Hub,Jones Beacon"
+ },
+ {
+ "name": "Unuteniling",
+ "pos_x": 168.28125,
+ "pos_y": -164.875,
+ "pos_z": -3.03125,
+ "stations": "Coggia Vision,Pajdusakova Terminal"
+ },
+ {
+ "name": "Unuteriges",
+ "pos_x": 148.46875,
+ "pos_y": -122.4375,
+ "pos_z": -59.375,
+ "stations": "Kawasato Colony"
+ },
+ {
+ "name": "Unutet",
+ "pos_x": 153.28125,
+ "pos_y": -131.0625,
+ "pos_z": 128.09375,
+ "stations": "Giunta Survey,Fox Point,Potagos Plant,Farouk Base"
+ },
+ {
+ "name": "Uodigaenta",
+ "pos_x": -1.28125,
+ "pos_y": -147.46875,
+ "pos_z": -98.6875,
+ "stations": "Mason Port,Michalitsianos Station,Daniel Beacon,Truly City"
+ },
+ {
+ "name": "Uodigi",
+ "pos_x": 50.03125,
+ "pos_y": -185.3125,
+ "pos_z": 1.09375,
+ "stations": "Argelander Hub,Bauman Enterprise,Brothers Silo,Nomura Vision,Dubyago Dock,Plante Relay"
+ },
+ {
+ "name": "Uodiomam",
+ "pos_x": 77.125,
+ "pos_y": -122.71875,
+ "pos_z": 171.46875,
+ "stations": "Siddha Stop"
+ },
+ {
+ "name": "Uolungh",
+ "pos_x": 161.59375,
+ "pos_y": -28.75,
+ "pos_z": 52.75,
+ "stations": "Lazutkin Relay,Hutton Mine"
+ },
+ {
+ "name": "Uolungura",
+ "pos_x": -92.53125,
+ "pos_y": -4.09375,
+ "pos_z": 68.28125,
+ "stations": "Popov Settlement,Ore Holdings,Bohr Station,Apt Relay"
+ },
+ {
+ "name": "Uoluskas",
+ "pos_x": 27.375,
+ "pos_y": 15.53125,
+ "pos_z": -94.28125,
+ "stations": "Curbeam Port,Herrington Dock,Moisuc Dock,Hubble Silo"
+ },
+ {
+ "name": "Upan",
+ "pos_x": -68.46875,
+ "pos_y": -83.21875,
+ "pos_z": 138.375,
+ "stations": "Midgeley Depot,Rozhdestvensky Relay"
+ },
+ {
+ "name": "Upane",
+ "pos_x": 45.90625,
+ "pos_y": -215.125,
+ "pos_z": 23.75,
+ "stations": "Wilson Prospect,Arkhangelsky Survey,Theodor Winnecke's Folly"
+ },
+ {
+ "name": "Upaniklis",
+ "pos_x": -151.21875,
+ "pos_y": -25,
+ "pos_z": 77.71875,
+ "stations": "Fozard Port,de Sousa Hub,Macdonald Platform,Piccard Penal colony"
+ },
+ {
+ "name": "Upannoda",
+ "pos_x": -15.96875,
+ "pos_y": 25.375,
+ "pos_z": 115.71875,
+ "stations": "Bohr Works"
+ },
+ {
+ "name": "Upem",
+ "pos_x": 207.96875,
+ "pos_y": -140.84375,
+ "pos_z": 46.0625,
+ "stations": "Fokker Point,Aitken Relay"
+ },
+ {
+ "name": "Upsilon Andromedae",
+ "pos_x": -30.4375,
+ "pos_y": -15.28125,
+ "pos_z": -27.90625,
+ "stations": "Moore City,Carlisle City,Dedman Bastion,Fidalgo Station"
+ },
+ {
+ "name": "Upsilon Aquarii",
+ "pos_x": -23.625,
+ "pos_y": -63.25,
+ "pos_z": 30.28125,
+ "stations": "Winne Station,Allen Hub,Hackworth Port,Jones Legacy"
+ },
+ {
+ "name": "Upsilon Phoenicis",
+ "pos_x": 47.1875,
+ "pos_y": -191.53125,
+ "pos_z": 16.15625,
+ "stations": "Extra Hub,Elgin Vision,Yerka Gateway,Mayor Installation"
+ },
+ {
+ "name": "Urabey",
+ "pos_x": 64.09375,
+ "pos_y": -18.71875,
+ "pos_z": -86.28125,
+ "stations": "Noon Landing"
+ },
+ {
+ "name": "Urabru",
+ "pos_x": 144.125,
+ "pos_y": -65.5,
+ "pos_z": -52.25,
+ "stations": "Vavrova Dock,Mobius Port,Fehrenbach Silo"
+ },
+ {
+ "name": "Uracaretii",
+ "pos_x": 100.71875,
+ "pos_y": 58,
+ "pos_z": -9.78125,
+ "stations": "Chamitoff Enterprise,Bass' Claim,Northrop Ring"
+ },
+ {
+ "name": "Uracenufon",
+ "pos_x": 166.71875,
+ "pos_y": 47.5,
+ "pos_z": 41.875,
+ "stations": "Bunch Dock,Cabral Landing,Gromov Dock,van Vogt Vista,Lee Mine"
+ },
+ {
+ "name": "Uram",
+ "pos_x": 44.28125,
+ "pos_y": 54.53125,
+ "pos_z": -3.4375,
+ "stations": "Oppenheimer Station,Atwater Refinery,Moon Base"
+ },
+ {
+ "name": "Urarina",
+ "pos_x": 57.25,
+ "pos_y": 106.75,
+ "pos_z": 32.96875,
+ "stations": "Ing Enterprise,Yamazaki Port,Haipeng Gateway,Moskowitz Silo"
+ },
+ {
+ "name": "Urcia",
+ "pos_x": 63.25,
+ "pos_y": -146.5,
+ "pos_z": -1.8125,
+ "stations": "Fedden City,Mikulin Dock,de Kamp Hub,Hadamard's Claim,Stevin Refinery,Martins Landing,Bond Port,de Kamp Port,Bailly Reach,Izumikawa Market,Evans Vision,Sitterly Vision,Maddox Depot,Henry Horizons,Millosevich Terminal"
+ },
+ {
+ "name": "Urd",
+ "pos_x": -85.28125,
+ "pos_y": -17.84375,
+ "pos_z": 78.59375,
+ "stations": "Britnev's Folly,Jemison Settlement"
+ },
+ {
+ "name": "Urhodiweu",
+ "pos_x": -74.53125,
+ "pos_y": -41.71875,
+ "pos_z": 68.3125,
+ "stations": "Bluford Settlement,de Andrade Point,Carson Enterprise"
+ },
+ {
+ "name": "Urhor",
+ "pos_x": 146.84375,
+ "pos_y": -42.375,
+ "pos_z": -74.4375,
+ "stations": "Oosterhoff Hub,Messier Survey"
+ },
+ {
+ "name": "Uroarbrunnr",
+ "pos_x": -57,
+ "pos_y": 85.28125,
+ "pos_z": 21.4375,
+ "stations": "Volkov Vision,Veblen Hub,Malcolm Vision,Clement Landing"
+ },
+ {
+ "name": "Uror",
+ "pos_x": 70.1875,
+ "pos_y": 77.6875,
+ "pos_z": -6.25,
+ "stations": "Arber Station"
+ },
+ {
+ "name": "Ursitoare",
+ "pos_x": -3.6875,
+ "pos_y": -60.8125,
+ "pos_z": -2.53125,
+ "stations": "Lucy Young's Orbital Happy Home,Milestones,Bond Beacon,Poisson Plant,Citroen Base"
+ },
+ {
+ "name": "Urthai",
+ "pos_x": 151.09375,
+ "pos_y": 13.3125,
+ "pos_z": 28.3125,
+ "stations": "Coats Vision,Sommerfeld City,Bushnell Station"
+ },
+ {
+ "name": "Urthingara",
+ "pos_x": 147.28125,
+ "pos_y": 26.40625,
+ "pos_z": -28.21875,
+ "stations": "Bobko Vision"
+ },
+ {
+ "name": "Uru",
+ "pos_x": -77.3125,
+ "pos_y": -99.625,
+ "pos_z": 18.5,
+ "stations": "Bessemer Vision,Stafford Gateway,Resnik Dock"
+ },
+ {
+ "name": "Urvane",
+ "pos_x": 83.5625,
+ "pos_y": -140.28125,
+ "pos_z": 38.8125,
+ "stations": "Lee Dock"
+ },
+ {
+ "name": "Urvantju",
+ "pos_x": -74.375,
+ "pos_y": -29.53125,
+ "pos_z": -61.09375,
+ "stations": "Onufrienko Terminal,Tavernier Gateway,Popovich Vision,Chadwick Survey"
+ },
+ {
+ "name": "Urvas",
+ "pos_x": -134.0625,
+ "pos_y": 4.875,
+ "pos_z": 85.28125,
+ "stations": "Robinson Station,Clement Silo,Bogdanov Stop"
+ },
+ {
+ "name": "Urvasini",
+ "pos_x": -104.78125,
+ "pos_y": -31.125,
+ "pos_z": -77.9375,
+ "stations": "Rae Dock,Baker Landing,Elder Settlement"
+ },
+ {
+ "name": "Usdia",
+ "pos_x": 133.9375,
+ "pos_y": -63.96875,
+ "pos_z": 81.28125,
+ "stations": "Fowler Orbital,Irens Relay,Opik Station,Velho Landing"
+ },
+ {
+ "name": "Usdiae",
+ "pos_x": 31.3125,
+ "pos_y": -142.0625,
+ "pos_z": -19.03125,
+ "stations": "Langsdorff Hub,Naburimannu Station,Forward Vision"
+ },
+ {
+ "name": "Usdian",
+ "pos_x": 111.0625,
+ "pos_y": 8.25,
+ "pos_z": 98.84375,
+ "stations": "Jeury Dock,Minkowski Silo"
+ },
+ {
+ "name": "Ushali",
+ "pos_x": 85.09375,
+ "pos_y": 15.03125,
+ "pos_z": -54.78125,
+ "stations": "Camarda Dock,Blaha Dock,Zoline Installation"
+ },
+ {
+ "name": "Usipedi",
+ "pos_x": 41.96875,
+ "pos_y": -22.5,
+ "pos_z": -114.21875,
+ "stations": "Ali Dock,Crampton Enterprise,Diamond Keep,Henslow Observatory"
+ },
+ {
+ "name": "Usipedia",
+ "pos_x": -74.09375,
+ "pos_y": 115.5,
+ "pos_z": 29.40625,
+ "stations": "Marlowe Dock,Ziemianski Survey,Menzies Settlement"
+ },
+ {
+ "name": "Usipediq",
+ "pos_x": 187.78125,
+ "pos_y": -128.03125,
+ "pos_z": -6.53125,
+ "stations": "Ziewe Vision,Adkins Port,Manning Vision"
+ },
+ {
+ "name": "Usipek",
+ "pos_x": 127.875,
+ "pos_y": -100,
+ "pos_z": 128.84375,
+ "stations": "Ernst Orbital,Reber Station"
+ },
+ {
+ "name": "Usiper",
+ "pos_x": 56.6875,
+ "pos_y": -237.125,
+ "pos_z": 40.03125,
+ "stations": "Ritchey Platform,al-Kashi Point"
+ },
+ {
+ "name": "Usiratiach",
+ "pos_x": -73.84375,
+ "pos_y": 46.5625,
+ "pos_z": 135.09375,
+ "stations": "Bhabha City,Pudwill Gorie Camp,Moriarty Survey"
+ },
+ {
+ "name": "Usiren",
+ "pos_x": 34.34375,
+ "pos_y": 38.84375,
+ "pos_z": 4.6875,
+ "stations": "Sinclair Platform,Diesel Terminal"
+ },
+ {
+ "name": "Uszaa",
+ "pos_x": 68.84375,
+ "pos_y": 48.75,
+ "pos_z": 74.75,
+ "stations": "Guest Installation,Patterson Dock,Rey Arsenal,Clauss Dock,Schouten Landing"
+ },
+ {
+ "name": "Utar",
+ "pos_x": 37.0625,
+ "pos_y": 91.625,
+ "pos_z": 20.71875,
+ "stations": "Watson Hub,Melroy City,Ivanchenkov Depot,Tesla City,Drexler Dock"
+ },
+ {
+ "name": "Uteran",
+ "pos_x": 93.15625,
+ "pos_y": 23.8125,
+ "pos_z": -14.0625,
+ "stations": "Walker Beacon,Ray Terminal,Asire Works"
+ },
+ {
+ "name": "Uterna",
+ "pos_x": -103.15625,
+ "pos_y": -115.25,
+ "pos_z": 88.6875,
+ "stations": "Shumil Dock"
+ },
+ {
+ "name": "Uterni",
+ "pos_x": 119.59375,
+ "pos_y": -59.46875,
+ "pos_z": -21.90625,
+ "stations": "Coulter Refinery,Hickman City,Kuchemann Port,Herzog Prospect,Beaumont Base"
+ },
+ {
+ "name": "Utero",
+ "pos_x": -37.125,
+ "pos_y": 33.71875,
+ "pos_z": -123.03125,
+ "stations": "Conway Terminal,Fernandes de Queiros' Folly"
+ },
+ {
+ "name": "Uteruku",
+ "pos_x": -54.8125,
+ "pos_y": 92.1875,
+ "pos_z": 59.15625,
+ "stations": "Smith Orbital,Liwei Base,Bethke Base"
+ },
+ {
+ "name": "Utgard",
+ "pos_x": 62.53125,
+ "pos_y": 76.125,
+ "pos_z": -29.375,
+ "stations": "Kubasov Dock,Bean Hub,Herrington Point"
+ },
+ {
+ "name": "Utgaroar",
+ "pos_x": -0.15625,
+ "pos_y": -102.75,
+ "pos_z": -5.5625,
+ "stations": "Fort Klarix"
+ },
+ {
+ "name": "Uthlan",
+ "pos_x": 136.15625,
+ "pos_y": -174.75,
+ "pos_z": -52.0625,
+ "stations": "Bailly Port"
+ },
+ {
+ "name": "Utse",
+ "pos_x": -31.53125,
+ "pos_y": 12.65625,
+ "pos_z": 73.53125,
+ "stations": "Whitworth Hangar,Yeliseyev Landing,Wolf Platform,Maire Base"
+ },
+ {
+ "name": "Utsegeri",
+ "pos_x": 90.90625,
+ "pos_y": -42.96875,
+ "pos_z": 148.6875,
+ "stations": "Proctor Survey,Wallis Enterprise,McCrea Dock,Balmer Base"
+ },
+ {
+ "name": "Utset",
+ "pos_x": 19.375,
+ "pos_y": -174.34375,
+ "pos_z": -82.15625,
+ "stations": "Hill Dock,Wilhelm von Struve Terminal,Sheckley Vision"
+ },
+ {
+ "name": "Utu",
+ "pos_x": 6.71875,
+ "pos_y": 46.15625,
+ "pos_z": 31.0625,
+ "stations": "Lubin Mines,Culpeper Port,Kondakova's Folly"
+ },
+ {
+ "name": "Uutagarama",
+ "pos_x": 150,
+ "pos_y": -8.8125,
+ "pos_z": -46.3125,
+ "stations": "McDivitt Terminal"
+ },
+ {
+ "name": "Uutasso",
+ "pos_x": 32.78125,
+ "pos_y": -29.96875,
+ "pos_z": 89.5625,
+ "stations": "Ejeta Station"
+ },
+ {
+ "name": "UX Arietis",
+ "pos_x": -53.25,
+ "pos_y": -64.09375,
+ "pos_z": -146.34375,
+ "stations": "Daglop Dock"
+ },
+ {
+ "name": "Uygur",
+ "pos_x": 105.21875,
+ "pos_y": -125.25,
+ "pos_z": 161.21875,
+ "stations": "Uto Depot,van der Riet Woolley Orbital,Frazetta Platform,White Reach"
+ },
+ {
+ "name": "Uygurr",
+ "pos_x": -156.90625,
+ "pos_y": 40.75,
+ "pos_z": 63.75,
+ "stations": "Bardeen Colony,Serebrov Station,Kovalevskaya Landing"
+ },
+ {
+ "name": "Uygurung",
+ "pos_x": 72.90625,
+ "pos_y": -76.34375,
+ "pos_z": 139.15625,
+ "stations": "Wilhelm von Struve Port"
+ },
+ {
+ "name": "Uzbekree",
+ "pos_x": -93.1875,
+ "pos_y": 36.6875,
+ "pos_z": 39.5625,
+ "stations": "Thuot Port,Armstrong City,Knight Base"
+ },
+ {
+ "name": "Uzumeru",
+ "pos_x": -37.90625,
+ "pos_y": -16.1875,
+ "pos_z": 52.84375,
+ "stations": "Dirac City,Lorentz Plant,Makarov Bastion"
+ },
+ {
+ "name": "Uzumo",
+ "pos_x": 21.90625,
+ "pos_y": -113.25,
+ "pos_z": -33.3125,
+ "stations": "Bohme Station,Arago Orbital,Cogswell Arsenal"
+ },
+ {
+ "name": "Uzumoku",
+ "pos_x": -95.21875,
+ "pos_y": 9.875,
+ "pos_z": -74.125,
+ "stations": "Buckell Station,Noguchi Base,Sverdrup Ring"
+ },
+ {
+ "name": "Uzumoxii",
+ "pos_x": -2.28125,
+ "pos_y": -33.15625,
+ "pos_z": -86.84375,
+ "stations": "Ferguson Ring"
+ },
+ {
+ "name": "V0972 Herculis",
+ "pos_x": -159.875,
+ "pos_y": 84.90625,
+ "pos_z": 97.5625,
+ "stations": "Malchiodi Escape,Doctorow Base"
+ },
+ {
+ "name": "V1082 Herculi",
+ "pos_x": -75.96875,
+ "pos_y": 68.21875,
+ "pos_z": 19.28125,
+ "stations": "Everest Hub,Bunnell Dock,George Enterprise,Abnett Barracks"
+ },
+ {
+ "name": "V1084 Tauri",
+ "pos_x": -16,
+ "pos_y": -27.90625,
+ "pos_z": -62.34375,
+ "stations": "Land Settlement,McMonagle Lab,Pythagoras Horizons"
+ },
+ {
+ "name": "V1090 Herculis",
+ "pos_x": -44.9375,
+ "pos_y": 36.9375,
+ "pos_z": 13.46875,
+ "stations": "Kaku Plant,Strekalov Depot"
+ },
+ {
+ "name": "V1198 Orionis",
+ "pos_x": 33.34375,
+ "pos_y": -45.9375,
+ "pos_z": -96.9375,
+ "stations": "Coats Colony,Carlisle Horizons,Anderson Horizons"
+ },
+ {
+ "name": "V1285 Aquilae",
+ "pos_x": -25.28125,
+ "pos_y": 1.625,
+ "pos_z": 28.84375,
+ "stations": "Kendrick Platform,Wait Colburn Installation,Tanner Plant"
+ },
+ {
+ "name": "V1581 Cygni",
+ "pos_x": -14.9375,
+ "pos_y": 2.25,
+ "pos_z": 2.9375,
+ "stations": "Peary Dock,Garan Hub"
+ },
+ {
+ "name": "V1688 Aquilae",
+ "pos_x": -61.9375,
+ "pos_y": -0.75,
+ "pos_z": 59.1875,
+ "stations": "Caselberg Orbital,Blaylock Enterprise,Littlewood Terminal,Fernandes de Queiros Ring,Eanes Gateway,Thompson Terminal,Levinson Station,Crown Enterprise,Rangarajan Port"
+ },
+ {
+ "name": "V1703 Aquilae",
+ "pos_x": -40.09375,
+ "pos_y": -20.71875,
+ "pos_z": 43.96875,
+ "stations": "Kafka Reach,Bosch Settlement,Houssay Plant"
+ },
+ {
+ "name": "V2151 Cygni",
+ "pos_x": -63.75,
+ "pos_y": 3.65625,
+ "pos_z": -5.59375,
+ "stations": "Eyharts Hub,Scott Vision"
+ },
+ {
+ "name": "V2160 Cygni",
+ "pos_x": -88.03125,
+ "pos_y": -19.28125,
+ "pos_z": 12,
+ "stations": "Reynolds Dock"
+ },
+ {
+ "name": "V2213 Ophiuchii",
+ "pos_x": -22.28125,
+ "pos_y": 26.53125,
+ "pos_z": 57.8125,
+ "stations": "de Andrade Dock,Compton Installation,Heinlein Terminal,Killough Port,Williams Hub"
+ },
+ {
+ "name": "V2431 Cygni",
+ "pos_x": -75.28125,
+ "pos_y": -10.21875,
+ "pos_z": 19.53125,
+ "stations": "Herschel Point"
+ },
+ {
+ "name": "V2578 Ophiuchii",
+ "pos_x": -2.15625,
+ "pos_y": 28.09375,
+ "pos_z": 64.28125,
+ "stations": "Bosch Station,Wedge Orbital,Drexler Station,Gutierrez Port,Freud Terminal,Rukavishnikov Market,de Caminha Holdings,Karlsefni Depot,Savery Hub,Okorafor Plant"
+ },
+ {
+ "name": "V2689 Orionis",
+ "pos_x": 8.90625,
+ "pos_y": -6.625,
+ "pos_z": -34.9375,
+ "stations": "Hodgson Dock,Capek Ring,Powell Ring,Wyndham Ring"
+ },
+ {
+ "name": "V350 Canis Majoris",
+ "pos_x": 137.78125,
+ "pos_y": -35.09375,
+ "pos_z": -105.6875,
+ "stations": "Back Colony,Scortia Survey"
+ },
+ {
+ "name": "V352 Canis Majoris",
+ "pos_x": 40.3125,
+ "pos_y": -16.9375,
+ "pos_z": -32.59375,
+ "stations": "Leonov Terminal,Rolland Holdings,Needham Gateway,Usachov Port"
+ },
+ {
+ "name": "V371 Normae",
+ "pos_x": 44.4375,
+ "pos_y": -6.53125,
+ "pos_z": 76.375,
+ "stations": "Reilly Gateway,Smith Enterprise,Phillips Gateway,Culbertson Hub,Artyukhin Gateway,Tani Port"
+ },
+ {
+ "name": "V372 Puppis",
+ "pos_x": 45.84375,
+ "pos_y": -5.09375,
+ "pos_z": -22.15625,
+ "stations": "Bowersox Gateway"
+ },
+ {
+ "name": "V374 Andromedae",
+ "pos_x": -39,
+ "pos_y": -15.9375,
+ "pos_z": -41.3125,
+ "stations": "Liska Station"
+ },
+ {
+ "name": "V374 Pegasi",
+ "pos_x": -26.90625,
+ "pos_y": -10.5625,
+ "pos_z": 2.90625,
+ "stations": "MacKellar Hub,Creamer Ring,Dorsett Dock,Debye Installation"
+ },
+ {
+ "name": "V379 Serpentis",
+ "pos_x": -1.40625,
+ "pos_y": 37.0625,
+ "pos_z": 35.9375,
+ "stations": "Meade Platform"
+ },
+ {
+ "name": "V390 Pavonis",
+ "pos_x": 42.46875,
+ "pos_y": -64.03125,
+ "pos_z": 64.46875,
+ "stations": "Youll Relay,Uto Platform,Yuzhe Refinery"
+ },
+ {
+ "name": "V397 Hydrae",
+ "pos_x": 47.375,
+ "pos_y": 26.28125,
+ "pos_z": -51.5625,
+ "stations": "Coats Point,Mendeleev Colony"
+ },
+ {
+ "name": "V401 Hydrae",
+ "pos_x": -82.15625,
+ "pos_y": -0.59375,
+ "pos_z": 1.75,
+ "stations": "Nobel Depot,Hinz Terminal,Swanwick Terminal"
+ },
+ {
+ "name": "V402 Pegasi",
+ "pos_x": -61.96875,
+ "pos_y": -19.375,
+ "pos_z": 4.9375,
+ "stations": "Leeuwenhoek Orbital,Silverberg Base,Georg Bothe City"
+ },
+ {
+ "name": "V405 Hydrae",
+ "pos_x": 78.0625,
+ "pos_y": 32.21875,
+ "pos_z": -37.21875,
+ "stations": "Tennyson d'Eyncourt Gateway"
+ },
+ {
+ "name": "V406 Pegasi",
+ "pos_x": -65.6875,
+ "pos_y": -37.5,
+ "pos_z": -17.65625,
+ "stations": "Vian Survey,Barry Station"
+ },
+ {
+ "name": "V417 Hydrae",
+ "pos_x": 61.65625,
+ "pos_y": 44,
+ "pos_z": -20.125,
+ "stations": "Bayliss Dock,Quick Vision,Hamilton Vision,Howard Horizons,de Sousa Installation,Ferguson Reach"
+ },
+ {
+ "name": "V418 Hydrae",
+ "pos_x": 82.125,
+ "pos_y": 64.34375,
+ "pos_z": -13.21875,
+ "stations": "Shunn Survey,Lovelace Depot"
+ },
+ {
+ "name": "V419 Hydrae",
+ "pos_x": 62.625,
+ "pos_y": 30.625,
+ "pos_z": 2.78125,
+ "stations": "Potter Orbital,Caidin Arsenal"
+ },
+ {
+ "name": "V443 Lacertae",
+ "pos_x": -108.59375,
+ "pos_y": -16.78125,
+ "pos_z": -8,
+ "stations": "Crouch Terminal,Clark Port"
+ },
+ {
+ "name": "V484 Tauri",
+ "pos_x": -0.625,
+ "pos_y": -18.28125,
+ "pos_z": -48.4375,
+ "stations": "Wnuk-Lipinski Orbital,Sterling Refinery"
+ },
+ {
+ "name": "V491 Persei",
+ "pos_x": -21.90625,
+ "pos_y": -11.25,
+ "pos_z": -61.78125,
+ "stations": "Rand City,Tavares Point,Ocampo Depot"
+ },
+ {
+ "name": "V492 Lyrae",
+ "pos_x": -40.96875,
+ "pos_y": 14.78125,
+ "pos_z": 15.0625,
+ "stations": "Bain Colony"
+ },
+ {
+ "name": "V538 Aurigae",
+ "pos_x": -14.25,
+ "pos_y": 8.625,
+ "pos_z": -36.4375,
+ "stations": "Wells Ring,Roddenberry Gateway,Koontz Dock"
+ },
+ {
+ "name": "V565 Persei",
+ "pos_x": -68.46875,
+ "pos_y": -10.40625,
+ "pos_z": -87.5625,
+ "stations": "Guest Terminal,Zamka Terminal,Przhevalsky Depot"
+ },
+ {
+ "name": "V590 Lyrae",
+ "pos_x": -98,
+ "pos_y": 41.75,
+ "pos_z": 54.84375,
+ "stations": "Wallace Terminal,Schwann Hangar,Bosch Vision"
+ },
+ {
+ "name": "V595 Lyrae",
+ "pos_x": -102.90625,
+ "pos_y": 34,
+ "pos_z": 41.78125,
+ "stations": "Willis Ring,Al-Kashi Dock,Lambert Park,Ramelli Enterprise"
+ },
+ {
+ "name": "V640 Cassiopeia",
+ "pos_x": -62.0625,
+ "pos_y": -4.53125,
+ "pos_z": -32.21875,
+ "stations": "Vardeman City,Ings Terminal,Conrad Arsenal,Lee City,O'Donnell Horizons,Fernandes de Queiros Orbital,Gelfand Vision,Akers Orbital,Galiano's Inheritance,Bellamy Dock,Bradbury City,Hennepin Reach,Lorrah Terminal,Caselberg Colony"
+ },
+ {
+ "name": "V711 Tauri",
+ "pos_x": 6.9375,
+ "pos_y": -65.625,
+ "pos_z": -75.28125,
+ "stations": "Potter Port,Guidoni Station,Collins Station"
+ },
+ {
+ "name": "V740 Cassiopeiae",
+ "pos_x": -86.1875,
+ "pos_y": 7.15625,
+ "pos_z": -47.78125,
+ "stations": "Bouch Orbital,Davy Station,Shepherd Terminal,Balandin Port,Pinzon Settlement"
+ },
+ {
+ "name": "V749 Herculis",
+ "pos_x": -41.53125,
+ "pos_y": 42.6875,
+ "pos_z": 46.96875,
+ "stations": "Pauling Gateway,Sarafanov Landing,Behnken Landing,Lawhead Legacy"
+ },
+ {
+ "name": "V774 Herculis",
+ "pos_x": -57.03125,
+ "pos_y": 17.28125,
+ "pos_z": 45.40625,
+ "stations": "Mendel Mines,Lazutkin Colony,Jacobi Asylum"
+ },
+ {
+ "name": "V774 Tauri",
+ "pos_x": 7.34375,
+ "pos_y": -34.6875,
+ "pos_z": -59.875,
+ "stations": "Grover Platform,Schirra Horizons"
+ },
+ {
+ "name": "V775 Herculis",
+ "pos_x": -56.34375,
+ "pos_y": 11.125,
+ "pos_z": 39.625,
+ "stations": "Vlamingh Hub,Beatty Port,Fontenay Depot,Cabot Installation,Vonarburg Enterprise"
+ },
+ {
+ "name": "V780 Tauri",
+ "pos_x": 1.6875,
+ "pos_y": -1.875,
+ "pos_z": -33.65625,
+ "stations": "Morris Station,Adams Colony"
+ },
+ {
+ "name": "V816 Herculis",
+ "pos_x": -23.5625,
+ "pos_y": 4.8125,
+ "pos_z": 24,
+ "stations": "Willis Installation,Hobaugh Hangar"
+ },
+ {
+ "name": "V841 Arae",
+ "pos_x": 58.53125,
+ "pos_y": -15.53125,
+ "pos_z": 102.125,
+ "stations": "Timofeyevich Terminal"
+ },
+ {
+ "name": "V848 Monocerotis",
+ "pos_x": 44,
+ "pos_y": -2.1875,
+ "pos_z": -91.3125,
+ "stations": "Antonelli Prospect,Russell Relay"
+ },
+ {
+ "name": "V857 Centauri",
+ "pos_x": 30.375,
+ "pos_y": 11.125,
+ "pos_z": 9.6875,
+ "stations": "Benz Port"
+ },
+ {
+ "name": "V886 Centauri",
+ "pos_x": 45.15625,
+ "pos_y": 12.125,
+ "pos_z": 26.96875,
+ "stations": "Garriott Terminal,Tanner City,Holdstock Silo"
+ },
+ {
+ "name": "V898 Herculi",
+ "pos_x": -63.15625,
+ "pos_y": 76.15625,
+ "pos_z": 59.90625,
+ "stations": "White Terminal,Sylvester Depot,Amundsen Camp"
+ },
+ {
+ "name": "V902 Centauri",
+ "pos_x": 83.90625,
+ "pos_y": 17.28125,
+ "pos_z": 26.59375,
+ "stations": "Olsen Hub,Berners-Lee Hub,Ali Station,Grimwood Exchange,Irvin Terminal,Shkaplerov Terminal,Perrin Dock,Bluford Dock,Chamitoff Hub,Holland Holdings,North Gateway,Lawhead's Claim"
+ },
+ {
+ "name": "V989 Cassiopeiae",
+ "pos_x": -45.375,
+ "pos_y": 6.78125,
+ "pos_z": -39.625,
+ "stations": "Tarelkin Refinery"
+ },
+ {
+ "name": "Vaccae",
+ "pos_x": 138.5,
+ "pos_y": -12.375,
+ "pos_z": -42.03125,
+ "stations": "Asaro Port,Cauchy Barracks,Lander Enterprise"
+ },
+ {
+ "name": "Vacha",
+ "pos_x": 149.03125,
+ "pos_y": 121.3125,
+ "pos_z": -59.46875,
+ "stations": "Andree Relay,Goulart's Claim"
+ },
+ {
+ "name": "Vacoch",
+ "pos_x": -34.21875,
+ "pos_y": -167.34375,
+ "pos_z": 72.0625,
+ "stations": "Dominique Port"
+ },
+ {
+ "name": "Vadi",
+ "pos_x": 152,
+ "pos_y": -93.09375,
+ "pos_z": 113.84375,
+ "stations": "Gurevich Beacon,Kosai Survey"
+ },
+ {
+ "name": "Vadimila",
+ "pos_x": 52.59375,
+ "pos_y": 19.875,
+ "pos_z": -154.8125,
+ "stations": "Laird Orbital,Moore Prospect,Khan Landing"
+ },
+ {
+ "name": "Vadimo",
+ "pos_x": 66.5625,
+ "pos_y": -62.53125,
+ "pos_z": 60.84375,
+ "stations": "Valz Beacon,Sikorsky Station,Lewis Holdings"
+ },
+ {
+ "name": "Vadimui",
+ "pos_x": -2.90625,
+ "pos_y": -144.09375,
+ "pos_z": 2.25,
+ "stations": "Lupoff's Folly"
+ },
+ {
+ "name": "Vaditaba",
+ "pos_x": 117.75,
+ "pos_y": 20.125,
+ "pos_z": -88.375,
+ "stations": "Lounge Station,Dezhurov Port,Hale Hub,Frechet Prospect"
+ },
+ {
+ "name": "Vaftetia",
+ "pos_x": 141.71875,
+ "pos_y": -195.1875,
+ "pos_z": 75.0625,
+ "stations": "Dahm Survey"
+ },
+ {
+ "name": "Vafthruva",
+ "pos_x": -90.84375,
+ "pos_y": -23.3125,
+ "pos_z": 99.375,
+ "stations": "Barnaby Hub,Gentle Platform,Payne Holdings,Andersson Beacon"
+ },
+ {
+ "name": "Vagha",
+ "pos_x": -39.84375,
+ "pos_y": -19.59375,
+ "pos_z": -118.15625,
+ "stations": "Piserchia Enterprise,Makeev Dock,Salak Bastion"
+ },
+ {
+ "name": "Vagho",
+ "pos_x": -100.21875,
+ "pos_y": 3.09375,
+ "pos_z": -60.25,
+ "stations": "Lounge Dock,Sturckow Port,Ferguson Enterprise,Illy Station,Curie Enterprise,Quinn's Progress,Lem's Pride,Turzillo Vision"
+ },
+ {
+ "name": "Vaipacnal",
+ "pos_x": 111.5625,
+ "pos_y": -168.25,
+ "pos_z": -37.6875,
+ "stations": "Michalitsianos' Exile,Youll's Progress"
+ },
+ {
+ "name": "Vaipacnali",
+ "pos_x": 120.40625,
+ "pos_y": -164.84375,
+ "pos_z": -79.875,
+ "stations": "Merritt Survey,Beshore's Claim,Montrose Works"
+ },
+ {
+ "name": "Vaipuna",
+ "pos_x": 132.53125,
+ "pos_y": -157.09375,
+ "pos_z": -45.40625,
+ "stations": "Mayer Enterprise"
+ },
+ {
+ "name": "Vaipurna",
+ "pos_x": 14.375,
+ "pos_y": -141.375,
+ "pos_z": 122.78125,
+ "stations": "Newcomb Holdings,Smoot Dock,Baillaud Station,Ziemianski Mine"
+ },
+ {
+ "name": "Vaiunia",
+ "pos_x": 16.40625,
+ "pos_y": -203.3125,
+ "pos_z": 43.59375,
+ "stations": "Schoenherr Terminal,Mattei Point"
+ },
+ {
+ "name": "Vaiuninguru",
+ "pos_x": 97.34375,
+ "pos_y": 92.8125,
+ "pos_z": -107.75,
+ "stations": "McMullen Settlement,Klein Orbital,Ogden Terminal"
+ },
+ {
+ "name": "Vaiunites",
+ "pos_x": 136.34375,
+ "pos_y": -113.4375,
+ "pos_z": 96.21875,
+ "stations": "Fedden Base,Piano Survey"
+ },
+ {
+ "name": "Vaiunito",
+ "pos_x": 76.125,
+ "pos_y": -171.4375,
+ "pos_z": 70.34375,
+ "stations": "Ahnert-Rohlfs Prospect,Gurevich Platform"
+ },
+ {
+ "name": "Vaiurisc",
+ "pos_x": 107.59375,
+ "pos_y": 18,
+ "pos_z": -15.1875,
+ "stations": "Robinson Dock,Land Dock,Adams Ring,Kolmogorov Enterprise,Scortia Terminal,Cummings Ring,White Port,McMahon Beacon,Vancouver Beacon"
+ },
+ {
+ "name": "Vaivares",
+ "pos_x": -98.6875,
+ "pos_y": 22.71875,
+ "pos_z": 102.71875,
+ "stations": "Gibson Point,Offutt Plant"
+ },
+ {
+ "name": "Vaizdiko",
+ "pos_x": 147.78125,
+ "pos_y": 28.625,
+ "pos_z": 55.125,
+ "stations": "Gubarev Landing,Titov Port,Pike Installation"
+ },
+ {
+ "name": "Vajrapem",
+ "pos_x": 81.1875,
+ "pos_y": -98.59375,
+ "pos_z": 159.65625,
+ "stations": "Marusek Plant,Perga Hub,MacLeod Platform,Rondon Orbital,Fremion Bastion"
+ },
+ {
+ "name": "Vajrapese",
+ "pos_x": 18.15625,
+ "pos_y": -141.84375,
+ "pos_z": 19.28125,
+ "stations": "Shajn Prospect,Kelly's Progress"
+ },
+ {
+ "name": "Vajrappa",
+ "pos_x": 89.8125,
+ "pos_y": 79.3125,
+ "pos_z": -129.4375,
+ "stations": "Wingqvist Station,Judson Arsenal,Abasheli Laboratory"
+ },
+ {
+ "name": "Vak",
+ "pos_x": 1.8125,
+ "pos_y": -90.90625,
+ "pos_z": 94.9375,
+ "stations": "Bothezat Horizons,Poisson Terminal"
+ },
+ {
+ "name": "Vaka",
+ "pos_x": 11.125,
+ "pos_y": -86.3125,
+ "pos_z": 39.125,
+ "stations": "Zenbei Orbital,White Point,Dufay Vision,Speke Beacon"
+ },
+ {
+ "name": "Valac",
+ "pos_x": -9535.6875,
+ "pos_y": -914.59375,
+ "pos_z": 19784.78125,
+ "stations": "Salted Womb"
+ },
+ {
+ "name": "Valda",
+ "pos_x": -92.34375,
+ "pos_y": -7.6875,
+ "pos_z": 47.8125,
+ "stations": "Kier Enterprise,Clairaut Dock,Wolfe Holdings,Rotsler Works,Noon Ring,Weil Settlement,Serre Dock,Bohnhoff Hub,Eilenberg Ring,Veblen Depot"
+ },
+ {
+ "name": "Valdawongol",
+ "pos_x": 18.75,
+ "pos_y": -13.4375,
+ "pos_z": -156.1875,
+ "stations": "Delbruck's Inheritance,Baudry Hub,Mach Port,Halsell Port,Smith's Progress"
+ },
+ {
+ "name": "Valder",
+ "pos_x": -119.59375,
+ "pos_y": -71.65625,
+ "pos_z": 79.40625,
+ "stations": "Macdonald Camp,Schmidt Platform,Clebsch Prospect"
+ },
+ {
+ "name": "Valdurchin",
+ "pos_x": 144.6875,
+ "pos_y": -119.375,
+ "pos_z": -39.46875,
+ "stations": "Valigursky Mines,Zhu Dock"
+ },
+ {
+ "name": "Valhaling",
+ "pos_x": -12.09375,
+ "pos_y": 77.96875,
+ "pos_z": 83.0625,
+ "stations": "Massimino Relay,Garn Settlement,Ferguson Prospect"
+ },
+ {
+ "name": "Valhoujem",
+ "pos_x": 84.46875,
+ "pos_y": -69.8125,
+ "pos_z": 65.15625,
+ "stations": "Challis Landing,Lemaitre Vision,Dugan Survey,Crown Camp"
+ },
+ {
+ "name": "Vali",
+ "pos_x": -124.4375,
+ "pos_y": 3.90625,
+ "pos_z": -63.4375,
+ "stations": "Al Saud Terminal,Henricks Holdings,Pogue Refinery"
+ },
+ {
+ "name": "Valimal",
+ "pos_x": -121,
+ "pos_y": -20.40625,
+ "pos_z": -15.96875,
+ "stations": "Gardner Terminal,Coppel Point,Nearchus Refinery"
+ },
+ {
+ "name": "Valis",
+ "pos_x": -78.59375,
+ "pos_y": -57.46875,
+ "pos_z": 118.03125,
+ "stations": "Mitchell Hub,Guidoni Survey,Blalock Station,Johnson Relay,Smith Penal colony"
+ },
+ {
+ "name": "Valishibon",
+ "pos_x": -2.25,
+ "pos_y": -118.21875,
+ "pos_z": 80.59375,
+ "stations": "Vavrova Mines,Le Guin Prospect,Fox Settlement,Wu Platform"
+ },
+ {
+ "name": "ValiTem",
+ "pos_x": -127.25,
+ "pos_y": 64.65625,
+ "pos_z": 87.65625,
+ "stations": "Fourier Survey,Watts Port,Cayley Depot"
+ },
+ {
+ "name": "Valki",
+ "pos_x": -37.96875,
+ "pos_y": -65.5625,
+ "pos_z": 55.1875,
+ "stations": "Antonio de Andrade Arena,Simmons Plant"
+ },
+ {
+ "name": "Valkindan",
+ "pos_x": 47.78125,
+ "pos_y": -141.78125,
+ "pos_z": -3.71875,
+ "stations": "Rogers Vision,de Kamp City"
+ },
+ {
+ "name": "Valkondan",
+ "pos_x": -20.40625,
+ "pos_y": 18.90625,
+ "pos_z": 116.5,
+ "stations": "Pawelczyk Installation,Liska Lab"
+ },
+ {
+ "name": "Valku",
+ "pos_x": 138.5625,
+ "pos_y": -155.0625,
+ "pos_z": -62.46875,
+ "stations": "Kondo Dock,Howe Enterprise,Bahcall Orbital"
+ },
+ {
+ "name": "Valkunth",
+ "pos_x": -174.53125,
+ "pos_y": -16.5625,
+ "pos_z": 10.75,
+ "stations": "Haarsma Terminal,Napier Beacon,Tayler Terminal"
+ },
+ {
+ "name": "Valkups",
+ "pos_x": 40.84375,
+ "pos_y": 8.46875,
+ "pos_z": -107.875,
+ "stations": "Maxwell Mines,Henry Dock,Carver Mines,Poindexter Holdings,Payette Beacon,Rescue Ship - Henry Dock"
+ },
+ {
+ "name": "Valmiki",
+ "pos_x": 119.03125,
+ "pos_y": -70.125,
+ "pos_z": -117.1875,
+ "stations": "Eschbach Beacon,Pinzon Installation"
+ },
+ {
+ "name": "Valta",
+ "pos_x": -101.3125,
+ "pos_y": 23.34375,
+ "pos_z": 23.90625,
+ "stations": "Conway Dock,Fourneyron Ring,Knapp Beacon,Deere Orbital"
+ },
+ {
+ "name": "Valtam",
+ "pos_x": -16.625,
+ "pos_y": -70.65625,
+ "pos_z": -126.9375,
+ "stations": "Dalton Terminal,Still Orbital,Stephenson Point,Volterra Base"
+ },
+ {
+ "name": "Valtamkin",
+ "pos_x": 152.71875,
+ "pos_y": -77.34375,
+ "pos_z": 105.09375,
+ "stations": "Friedrich Peters Terminal,Perlmutter Station,Lozino-Lozinskiy Gateway,Reynolds Survey,Fourier Horizons"
+ },
+ {
+ "name": "Valtani",
+ "pos_x": -15.3125,
+ "pos_y": -15.4375,
+ "pos_z": -179.9375,
+ "stations": "Akers Mine,Gann Dock"
+ },
+ {
+ "name": "Valteki",
+ "pos_x": 41.90625,
+ "pos_y": -46.375,
+ "pos_z": -65.15625,
+ "stations": "Thoreau Platform"
+ },
+ {
+ "name": "Valtys",
+ "pos_x": -35.90625,
+ "pos_y": -63.375,
+ "pos_z": -60.90625,
+ "stations": "Oleskiw City,Patterson Base,Veach Dock,Sharman Dock,Auld Colony,Hoshide Base"
+ },
+ {
+ "name": "Vamm",
+ "pos_x": 52.6875,
+ "pos_y": 78.28125,
+ "pos_z": 23.6875,
+ "stations": "Tanner Port,McNair Ring,Elion Terminal,Lavrador Base,Nelson Barracks,Nemere's Inheritance"
+ },
+ {
+ "name": "van Maanen's Star",
+ "pos_x": -6.3125,
+ "pos_y": -11.6875,
+ "pos_z": -4.125,
+ "stations": "O'Connor City,Jeury Terminal,Thurston Gateway,Bierce Survey"
+ },
+ {
+ "name": "Vana",
+ "pos_x": -0.65625,
+ "pos_y": -68.53125,
+ "pos_z": 114.84375,
+ "stations": "Sternbach Landing"
+ },
+ {
+ "name": "Vanaharan",
+ "pos_x": 18.53125,
+ "pos_y": 36.4375,
+ "pos_z": 134.9375,
+ "stations": "Saberhagen Station,Williams Orbital,Sabine Terminal,Banach Station,Jenkinson Station,Ramsay Plant,Kratman Station,Verne Port,Stephens' Progress,Carstensz Base"
+ },
+ {
+ "name": "Vanawa",
+ "pos_x": -19.875,
+ "pos_y": 15.40625,
+ "pos_z": 111.75,
+ "stations": "Ostwald Platform,Xiaoguan Station,Worden Landing"
+ },
+ {
+ "name": "Vanayeb Hua",
+ "pos_x": -31.59375,
+ "pos_y": 152.59375,
+ "pos_z": -43.84375,
+ "stations": "Linaweaver Survey"
+ },
+ {
+ "name": "Vanayequi",
+ "pos_x": -88.5,
+ "pos_y": -12.3125,
+ "pos_z": 98.84375,
+ "stations": "Clauss Hub,Obruchev Port,Haldeman II Dock,Tavernier Orbital,Christian Installation,Lobachevsky City,Meredith Hub,Iqbal Settlement,King Orbital,Blackwell Point"
+ },
+ {
+ "name": "Vandar",
+ "pos_x": 48.78125,
+ "pos_y": 33.84375,
+ "pos_z": -78.8125,
+ "stations": "Wheelock Colony"
+ },
+ {
+ "name": "Vanir",
+ "pos_x": 0.78125,
+ "pos_y": -59.34375,
+ "pos_z": 40.625,
+ "stations": "Rennie Orbital,McClintock Dock,Farkas Keep,Leckie Vision,Ferguson Orbital,Lavoisier Relay"
+ },
+ {
+ "name": "Varam",
+ "pos_x": -60,
+ "pos_y": 132.0625,
+ "pos_z": 15.65625,
+ "stations": "Zebrowski Enterprise,Hippalus Enterprise,Phillips Mines,Malocello Prospect,Witt's Inheritance"
+ },
+ {
+ "name": "Varani",
+ "pos_x": 77.53125,
+ "pos_y": -43.65625,
+ "pos_z": 117.8125,
+ "stations": "Friedrich Peters Dock,Zetford Holdings,Hill Hub,Hoyle Landing,Gaultier de Varennes Base"
+ },
+ {
+ "name": "Varati",
+ "pos_x": -178.65625,
+ "pos_y": 77.125,
+ "pos_z": -87.125,
+ "stations": "Thompson Dock,Bond Hub,Remec Point,Forsskal Hub"
+ },
+ {
+ "name": "Varayah",
+ "pos_x": 52.75,
+ "pos_y": 16.75,
+ "pos_z": -9.875,
+ "stations": "Lind Terminal,Euler City,Artsebarsky Gateway,Tilman Survey"
+ },
+ {
+ "name": "Varchi",
+ "pos_x": -108.15625,
+ "pos_y": -81.15625,
+ "pos_z": 116.28125,
+ "stations": "Forbes' Exile,Grego Survey"
+ },
+ {
+ "name": "Vard",
+ "pos_x": 121.84375,
+ "pos_y": -34.21875,
+ "pos_z": -18.9375,
+ "stations": "Hoyle Depot,Asimov Vision,Hinz Outpost"
+ },
+ {
+ "name": "Vardh",
+ "pos_x": -34.21875,
+ "pos_y": -18.4375,
+ "pos_z": -113.8125,
+ "stations": "Phillips Survey,Reightler Relay,Archimedes Point"
+ },
+ {
+ "name": "Vardine",
+ "pos_x": 51.09375,
+ "pos_y": 98.75,
+ "pos_z": -116.84375,
+ "stations": "Lenthall Platform"
+ },
+ {
+ "name": "Varduli",
+ "pos_x": -68.28125,
+ "pos_y": -69.1875,
+ "pos_z": 151.5,
+ "stations": "Shinn Beacon,Martin Point"
+ },
+ {
+ "name": "Vargerson",
+ "pos_x": 95.53125,
+ "pos_y": -164.625,
+ "pos_z": 39.90625,
+ "stations": "Browncoat Refuge"
+ },
+ {
+ "name": "Varlawoth",
+ "pos_x": 102.1875,
+ "pos_y": -182.09375,
+ "pos_z": -84.53125,
+ "stations": "Hartmann Mine"
+ },
+ {
+ "name": "Varli",
+ "pos_x": 63.25,
+ "pos_y": 6.15625,
+ "pos_z": 120.03125,
+ "stations": "Gordon City,Qureshi Enterprise,Thomas Survey"
+ },
+ {
+ "name": "Varpas",
+ "pos_x": 56,
+ "pos_y": 153.90625,
+ "pos_z": -43.9375,
+ "stations": "Favier Settlement,Alexandrov Base"
+ },
+ {
+ "name": "Varpet",
+ "pos_x": 84.8125,
+ "pos_y": -20.90625,
+ "pos_z": -1.40625,
+ "stations": "Gaiman Station,Klink Station,Euler Installation,Abel Survey"
+ },
+ {
+ "name": "Varrala",
+ "pos_x": 18.96875,
+ "pos_y": 0.4375,
+ "pos_z": -159.75,
+ "stations": "Kaleri Port,Stafford Settlement"
+ },
+ {
+ "name": "Varramool",
+ "pos_x": 8,
+ "pos_y": 160.375,
+ "pos_z": -12.96875,
+ "stations": "Levy Hangar"
+ },
+ {
+ "name": "Varri",
+ "pos_x": 57.46875,
+ "pos_y": -169.5625,
+ "pos_z": -17.375,
+ "stations": "Cyrrhus Works"
+ },
+ {
+ "name": "Vasa",
+ "pos_x": -140.75,
+ "pos_y": -48.28125,
+ "pos_z": 85.59375,
+ "stations": "Sinclair Platform,Lee Camp"
+ },
+ {
+ "name": "Vasak",
+ "pos_x": 29.625,
+ "pos_y": -49.03125,
+ "pos_z": -126.71875,
+ "stations": "Lee Port,Rolland Port,Charnas Port,Bulmer Port,Jacobi Enterprise,Manning Dock,Anderson Terminal,McCarthy's Inheritance,Kandel Landing,Hedin Settlement,Alten Survey"
+ },
+ {
+ "name": "Vash",
+ "pos_x": 112.1875,
+ "pos_y": -26.78125,
+ "pos_z": 118.90625,
+ "stations": "Libeskind Settlement,Bond Bastion"
+ },
+ {
+ "name": "Vashalya",
+ "pos_x": -120.75,
+ "pos_y": -0.4375,
+ "pos_z": 37,
+ "stations": "Fernandes de Queiros Landing,Kennedy Base,Russell Prospect,Barentsz's Progress"
+ },
+ {
+ "name": "Vashis",
+ "pos_x": 107.4375,
+ "pos_y": 13.28125,
+ "pos_z": 0.4375,
+ "stations": "Williams Port,Bounds Port,Macan Camp,Gentle Hub,Fu Prospect,Stabenow's Folly"
+ },
+ {
+ "name": "Vashishtha",
+ "pos_x": 101.3125,
+ "pos_y": -70.53125,
+ "pos_z": 35.75,
+ "stations": "Stone Dock"
+ },
+ {
+ "name": "Vasho",
+ "pos_x": 125.0625,
+ "pos_y": -169.46875,
+ "pos_z": 157.59375,
+ "stations": "Robert Terminal,Abel Port,Kirby Dock,Hayashi Terminal"
+ },
+ {
+ "name": "Vasing",
+ "pos_x": 122.5,
+ "pos_y": -41.875,
+ "pos_z": -89.03125,
+ "stations": "Barratt Platform,Atkov Orbital,Pudwill Gorie Installation,Hausdorff's Folly"
+ },
+ {
+ "name": "Vasir",
+ "pos_x": -93.15625,
+ "pos_y": 75.84375,
+ "pos_z": 13.125,
+ "stations": "Reynolds Colony,Bacon Outpost"
+ },
+ {
+ "name": "Vasistha",
+ "pos_x": 89.375,
+ "pos_y": 24.65625,
+ "pos_z": -62.75,
+ "stations": "Emshwiller Landing,Steele Settlement"
+ },
+ {
+ "name": "Vasude",
+ "pos_x": 88.96875,
+ "pos_y": 19.46875,
+ "pos_z": -51.28125,
+ "stations": "Alexander Freeport,Diophantus Arsenal,Phillips Outpost"
+ },
+ {
+ "name": "Vasudevas",
+ "pos_x": 126.375,
+ "pos_y": 60.90625,
+ "pos_z": 42.03125,
+ "stations": "Viete Terminal"
+ },
+ {
+ "name": "Vasuki",
+ "pos_x": 101.40625,
+ "pos_y": -239.71875,
+ "pos_z": 51.25,
+ "stations": "Wasden Orbital"
+ },
+ {
+ "name": "Vasukili",
+ "pos_x": 166.03125,
+ "pos_y": -101.15625,
+ "pos_z": -18.28125,
+ "stations": "Roche Colony"
+ },
+ {
+ "name": "Vasupalinni",
+ "pos_x": -67.09375,
+ "pos_y": 75.59375,
+ "pos_z": 127.40625,
+ "stations": "Greenland Vision,Stevens' Exile,Chalker Terminal"
+ },
+ {
+ "name": "Vasupari",
+ "pos_x": 169.9375,
+ "pos_y": -69.125,
+ "pos_z": -57.34375,
+ "stations": "Hughes Platform,Phillips' Progress"
+ },
+ {
+ "name": "Vayurathi",
+ "pos_x": 70.21875,
+ "pos_y": -90.65625,
+ "pos_z": 100.125,
+ "stations": "Auld Point,Lopez Terminal,Allen St. John Dock"
+ },
+ {
+ "name": "Veami Kians",
+ "pos_x": 158.84375,
+ "pos_y": -130.1875,
+ "pos_z": 17.5625,
+ "stations": "Penrose's Progress,Wasden Prospect,Dowty Station"
+ },
+ {
+ "name": "Veamit",
+ "pos_x": 43.65625,
+ "pos_y": -133.5625,
+ "pos_z": -9.90625,
+ "stations": "Hatanaka Enterprise,van Rhijn Hub,Christy Point"
+ },
+ {
+ "name": "Vedi Di",
+ "pos_x": 16.46875,
+ "pos_y": -36.59375,
+ "pos_z": 127.8125,
+ "stations": "Hui Horizons"
+ },
+ {
+ "name": "Veditja",
+ "pos_x": 109.625,
+ "pos_y": -83.6875,
+ "pos_z": -65.84375,
+ "stations": "Pennington Ring"
+ },
+ {
+ "name": "Vega",
+ "pos_x": -21.90625,
+ "pos_y": 8.125,
+ "pos_z": 9,
+ "stations": "Taylor City,Fort Dixon,Budrys Terminal,Mori Terminal,Locke Terminal,Tarelkin Keep,Yu Relay,Mendez Horizons"
+ },
+ {
+ "name": "Veja Deng",
+ "pos_x": -12.53125,
+ "pos_y": -119.84375,
+ "pos_z": -92.21875,
+ "stations": "Roosa Terminal,Shull Gateway,Schweinfurth's Claim,Feoktistov Port"
+ },
+ {
+ "name": "Veja Manto",
+ "pos_x": -38.5625,
+ "pos_y": -94.5,
+ "pos_z": 46.53125,
+ "stations": "Farrer Terminal,Hope Platform"
+ },
+ {
+ "name": "Veja Moco",
+ "pos_x": 20.375,
+ "pos_y": -165.28125,
+ "pos_z": 23.25,
+ "stations": "Hampson Port,Neugebauer Vision"
+ },
+ {
+ "name": "Velabiani",
+ "pos_x": -52.6875,
+ "pos_y": -133.34375,
+ "pos_z": 13.1875,
+ "stations": "Titius Platform,Zander Settlement"
+ },
+ {
+ "name": "Velabri",
+ "pos_x": 66.375,
+ "pos_y": -41.46875,
+ "pos_z": 25.4375,
+ "stations": "Baade Port"
+ },
+ {
+ "name": "Velao",
+ "pos_x": 52.75,
+ "pos_y": 158.0625,
+ "pos_z": 30.09375,
+ "stations": "Goeschke Base,Wang Platform"
+ },
+ {
+ "name": "Velas",
+ "pos_x": 124.09375,
+ "pos_y": 89.1875,
+ "pos_z": 94.90625,
+ "stations": "Sohl Installation,Tarentum Station,Hamilton City"
+ },
+ {
+ "name": "Velebes",
+ "pos_x": -144.71875,
+ "pos_y": -89.65625,
+ "pos_z": 96.28125,
+ "stations": "Beaumont Depot"
+ },
+ {
+ "name": "Veleda",
+ "pos_x": -73,
+ "pos_y": -60.03125,
+ "pos_z": -131.75,
+ "stations": "Knight Mine,Robinson Survey"
+ },
+ {
+ "name": "Veletanyan",
+ "pos_x": -90.65625,
+ "pos_y": 18.375,
+ "pos_z": -161,
+ "stations": "Wylie Outpost,Eschbach Settlement"
+ },
+ {
+ "name": "Veleti",
+ "pos_x": -63.3125,
+ "pos_y": 99.03125,
+ "pos_z": -108.8125,
+ "stations": "Griffin Ring,Harrison Barracks"
+ },
+ {
+ "name": "Veli",
+ "pos_x": -83.375,
+ "pos_y": 136,
+ "pos_z": -21.21875,
+ "stations": "Pratchett Port,Gabriel Survey,Ellis Laboratory"
+ },
+ {
+ "name": "Veliep",
+ "pos_x": 96.3125,
+ "pos_y": -215.9375,
+ "pos_z": 70.78125,
+ "stations": "Roed Odegaard Enterprise,Qushji Survey,Kennicott Base"
+ },
+ {
+ "name": "Velim",
+ "pos_x": -0.5,
+ "pos_y": 81.5625,
+ "pos_z": 31.625,
+ "stations": "Bunnell Port,Tsunenaga Plant"
+ },
+ {
+ "name": "Velingai",
+ "pos_x": -30.25,
+ "pos_y": -2.78125,
+ "pos_z": 85.6875,
+ "stations": "Hennen Stop,Low Observatory"
+ },
+ {
+ "name": "Velis",
+ "pos_x": -131.65625,
+ "pos_y": -63.125,
+ "pos_z": 10.9375,
+ "stations": "Menezes Dock,Bao Dock"
+ },
+ {
+ "name": "Vellamo",
+ "pos_x": 16.78125,
+ "pos_y": 27.6875,
+ "pos_z": 72.625,
+ "stations": "Conrad Terminal,Dyr Horizons,Gubarev Mines"
+ },
+ {
+ "name": "Vellenu",
+ "pos_x": -145.5625,
+ "pos_y": 115.5,
+ "pos_z": 61.71875,
+ "stations": "Lukyanenko Settlement,Betancourt Bastion"
+ },
+ {
+ "name": "Velli",
+ "pos_x": 118.75,
+ "pos_y": -14.8125,
+ "pos_z": -49.71875,
+ "stations": "Brandenstein Mine"
+ },
+ {
+ "name": "Velliep",
+ "pos_x": 84.4375,
+ "pos_y": -59.03125,
+ "pos_z": 28.21875,
+ "stations": "Ikeya Plant,Giancola Installation,Hovgaard Landing,Flammarion Installation,Debehogne Hub"
+ },
+ {
+ "name": "Velnambang",
+ "pos_x": 101.8125,
+ "pos_y": -167.3125,
+ "pos_z": 52.03125,
+ "stations": "Koishikawa Gateway,Zhukovsky Port,Sawyer Survey,Brillant Relay"
+ },
+ {
+ "name": "Velnambe",
+ "pos_x": 105.84375,
+ "pos_y": -151.3125,
+ "pos_z": 97.5,
+ "stations": "Henderson Ring,Sitterly Ring,Volterra Depot,Galiano Horizons,Tully's Pride,Wells Palace,Bruck Vision"
+ },
+ {
+ "name": "Velnambo",
+ "pos_x": -53.4375,
+ "pos_y": 119.5625,
+ "pos_z": 35.75,
+ "stations": "Nelson Relay,Laue Terminal"
+ },
+ {
+ "name": "Velnians",
+ "pos_x": 80.96875,
+ "pos_y": 18.09375,
+ "pos_z": -101,
+ "stations": "Piercy Dock,West Market"
+ },
+ {
+ "name": "Velniassee",
+ "pos_x": 61.625,
+ "pos_y": -78.0625,
+ "pos_z": 146.0625,
+ "stations": "Lewis Colony"
+ },
+ {
+ "name": "Velniat",
+ "pos_x": 174.84375,
+ "pos_y": 7.84375,
+ "pos_z": 12.1875,
+ "stations": "Stephenson Hub,Zetford's Progress"
+ },
+ {
+ "name": "Venda Kono",
+ "pos_x": 53.59375,
+ "pos_y": 6.8125,
+ "pos_z": 122.03125,
+ "stations": "Elvstrom Legacy,Rond d'Alembert Port,Teng Relay"
+ },
+ {
+ "name": "Vendarid",
+ "pos_x": -56.78125,
+ "pos_y": -58.9375,
+ "pos_z": 143.5625,
+ "stations": "Macedo City,Soddy Installation,Gaspar de Portola Hub"
+ },
+ {
+ "name": "Vendeges",
+ "pos_x": 96.875,
+ "pos_y": -220.9375,
+ "pos_z": -20.5625,
+ "stations": "Derekas' Claim"
+ },
+ {
+ "name": "Vendelia",
+ "pos_x": -89.875,
+ "pos_y": 20.9375,
+ "pos_z": 126.75,
+ "stations": "Eddington Port"
+ },
+ {
+ "name": "Vendemo",
+ "pos_x": -25.9375,
+ "pos_y": -65.8125,
+ "pos_z": -36.46875,
+ "stations": "Ejeta Ring"
+ },
+ {
+ "name": "Venegana",
+ "pos_x": -18.65625,
+ "pos_y": -83.625,
+ "pos_z": 19.46875,
+ "stations": "Fettman Market,Foreman Gateway,Shull Ring,Grover Terminal,Laval Station,Gohar Hub,Crown Terminal"
+ },
+ {
+ "name": "Veneres",
+ "pos_x": -85.6875,
+ "pos_y": -116.875,
+ "pos_z": 0.1875,
+ "stations": "Kendrick Works,Faris Vision"
+ },
+ {
+ "name": "Veneri",
+ "pos_x": 35.6875,
+ "pos_y": 54.125,
+ "pos_z": 20.09375,
+ "stations": "Bombelli's Folly,al-Khayyam Hub,Toll Platform"
+ },
+ {
+ "name": "Venet",
+ "pos_x": 114.15625,
+ "pos_y": -109.96875,
+ "pos_z": 83.6875,
+ "stations": "Bradley Platform,Tan Vision,Barcelona Prospect,Drake Depot,Penzias Landing"
+ },
+ {
+ "name": "Venetet",
+ "pos_x": 78.40625,
+ "pos_y": -83.96875,
+ "pos_z": 69.25,
+ "stations": "Harding Vision,Sitter Holdings,Eisinga Ring,Hoyle's Progress,Westerhout Lab,Crossfield Keep"
+ },
+ {
+ "name": "Venetic",
+ "pos_x": -68.4375,
+ "pos_y": 14.8125,
+ "pos_z": 35.0625,
+ "stations": "Watson Colony,Boulton Station,Laplace Landing,Bergerac Gateway"
+ },
+ {
+ "name": "Venexo",
+ "pos_x": 128.09375,
+ "pos_y": -53.59375,
+ "pos_z": -2.125,
+ "stations": "Gaensler Dock,van der Riet Woolley Terminal,Cowling Orbital,Garfinkle Base,Lindblad Hub,van der Riet Woolley Orbital,Ludwig Struve Orbital,Youll Orbital,Hennepin Works,Mechain Vision,Steakley Observatory"
+ },
+ {
+ "name": "Venici",
+ "pos_x": 52.3125,
+ "pos_y": -201.53125,
+ "pos_z": -52.78125,
+ "stations": "Wolfe Depot,McManus Colony,Dixon Terminal,Jean Oasis"
+ },
+ {
+ "name": "Venicones",
+ "pos_x": -121.625,
+ "pos_y": -57,
+ "pos_z": 29.1875,
+ "stations": "Naddoddur Plant,Narvaez Settlement"
+ },
+ {
+ "name": "Venki",
+ "pos_x": -115.09375,
+ "pos_y": -102.65625,
+ "pos_z": 8.84375,
+ "stations": "Phillips Freeport,Acharya Prospect,Galiano Dock"
+ },
+ {
+ "name": "Venne",
+ "pos_x": 60.21875,
+ "pos_y": 33.59375,
+ "pos_z": -3.28125,
+ "stations": "Denton Enterprise,Baudin Hub"
+ },
+ {
+ "name": "Vennik",
+ "pos_x": -52.0625,
+ "pos_y": 127.53125,
+ "pos_z": 26.21875,
+ "stations": "Tarentum City,Nixon Enterprise,He Hub,Maine Hub"
+ },
+ {
+ "name": "Venux",
+ "pos_x": 57.09375,
+ "pos_y": -38.4375,
+ "pos_z": -52.96875,
+ "stations": "Hughes-Fulford Dock,Weil Point"
+ },
+ {
+ "name": "Vequess",
+ "pos_x": 62.5625,
+ "pos_y": -106,
+ "pos_z": 22.125,
+ "stations": "Agnews' Folly,Cuffey Orbital"
+ },
+ {
+ "name": "Veramonawe",
+ "pos_x": 23.8125,
+ "pos_y": -121,
+ "pos_z": 121.1875,
+ "stations": "Lee Ring,Leonard Vision,Bailly Port,Grassmann's Folly"
+ },
+ {
+ "name": "Veratha",
+ "pos_x": -20.34375,
+ "pos_y": 123.0625,
+ "pos_z": -126,
+ "stations": "Sadi Carnot Terminal,Clement Depot,Ewald Point"
+ },
+ {
+ "name": "Verbi",
+ "pos_x": 15.65625,
+ "pos_y": -211.3125,
+ "pos_z": 9.71875,
+ "stations": "Sandage Landing,Araki Terminal,Jones Vision,Froud's Inheritance"
+ },
+ {
+ "name": "Verbigeni",
+ "pos_x": -64.75,
+ "pos_y": -7.6875,
+ "pos_z": 57,
+ "stations": "Archambault Plant"
+ },
+ {
+ "name": "Verbigenses",
+ "pos_x": 95.9375,
+ "pos_y": -198,
+ "pos_z": 15.75,
+ "stations": "Hariot's Claim"
+ },
+ {
+ "name": "Verdia",
+ "pos_x": -50.8125,
+ "pos_y": -148.8125,
+ "pos_z": -35.25,
+ "stations": "Giclas Prospect,Hampson's Inheritance"
+ },
+ {
+ "name": "Verner",
+ "pos_x": 74.1875,
+ "pos_y": -47.09375,
+ "pos_z": -10.03125,
+ "stations": "Fokker Penal colony,Littrow Vision,Jael Dock,SJ Parkinson"
+ },
+ {
+ "name": "Veroa",
+ "pos_x": 49.09375,
+ "pos_y": 34.28125,
+ "pos_z": -74.9375,
+ "stations": "Lunan Landing"
+ },
+ {
+ "name": "Veroandi",
+ "pos_x": 33.15625,
+ "pos_y": -37.625,
+ "pos_z": 32.25,
+ "stations": "Vardeman Gateway,Panshin Hub,Henson Station,Gromov Orbital,Baker Orbital,Heinkel Settlement,Simmons Settlement"
+ },
+ {
+ "name": "Veroklist",
+ "pos_x": 47.3125,
+ "pos_y": -260.09375,
+ "pos_z": 73.5625,
+ "stations": "Vaucouleurs Vision"
+ },
+ {
+ "name": "Veroo",
+ "pos_x": 23.5,
+ "pos_y": -18.75,
+ "pos_z": 146.875,
+ "stations": "Hunziker Terminal,Jernigan City,Behnken Enterprise,Gotlieb Enterprise,Elion Arsenal"
+ },
+ {
+ "name": "Verovichili",
+ "pos_x": 146.46875,
+ "pos_y": 0.28125,
+ "pos_z": 65.40625,
+ "stations": "Gibson Gateway,Blackwell City,Napier Enterprise"
+ },
+ {
+ "name": "Vertam",
+ "pos_x": -64.65625,
+ "pos_y": -7.90625,
+ "pos_z": -165.75,
+ "stations": "Chios Settlement,Scheerbart Keep,Fernandes Installation"
+ },
+ {
+ "name": "Vertamocori",
+ "pos_x": -26.9375,
+ "pos_y": 63.1875,
+ "pos_z": -132.4375,
+ "stations": "Clement Orbital,Curie Station"
+ },
+ {
+ "name": "Vertarindji",
+ "pos_x": 125.625,
+ "pos_y": -108.90625,
+ "pos_z": 109.46875,
+ "stations": "Meinel City,Hussenot Landing,Sabine Installation"
+ },
+ {
+ "name": "Vertheli",
+ "pos_x": 45.53125,
+ "pos_y": 125.78125,
+ "pos_z": -24.375,
+ "stations": "Irvin Camp"
+ },
+ {
+ "name": "Vertnyi",
+ "pos_x": 138.5,
+ "pos_y": -219.90625,
+ "pos_z": -11.875,
+ "stations": "Longyear Enterprise,Banneker Platform,Mayer Orbital"
+ },
+ {
+ "name": "Vertserus",
+ "pos_x": -142.09375,
+ "pos_y": 4.25,
+ "pos_z": -9.25,
+ "stations": "Fung's Claim,Almagro Plant,Crouch Platform"
+ },
+ {
+ "name": "Vertsety",
+ "pos_x": 108.5,
+ "pos_y": -28.875,
+ "pos_z": 78.28125,
+ "stations": "Anderson Platform"
+ },
+ {
+ "name": "Vertumnus",
+ "pos_x": -54.28125,
+ "pos_y": 13.875,
+ "pos_z": 19.40625,
+ "stations": "Rawn City"
+ },
+ {
+ "name": "VESPER-M4",
+ "pos_x": -3.6875,
+ "pos_y": -22.53125,
+ "pos_z": 57,
+ "stations": "Slough Orbital,Rothfuss Holdings,Oluwafemi Depot"
+ },
+ {
+ "name": "Vestani",
+ "pos_x": 45.6875,
+ "pos_y": 98.53125,
+ "pos_z": 56.9375,
+ "stations": "Rose Terminal,Tapinas Hub,Chorel Silo"
+ },
+ {
+ "name": "Vestet",
+ "pos_x": -73.1875,
+ "pos_y": -119.03125,
+ "pos_z": -109.03125,
+ "stations": "Goldstein Freeport"
+ },
+ {
+ "name": "Vesui",
+ "pos_x": 160.0625,
+ "pos_y": -1.28125,
+ "pos_z": -8.53125,
+ "stations": "Kirchoff Terminal,Rodrigues Reach"
+ },
+ {
+ "name": "Vesuvit",
+ "pos_x": 145.09375,
+ "pos_y": -239.5625,
+ "pos_z": 97.4375,
+ "stations": "Suri Gateway,Miyasaka Mines,Fozard Vision,Robinson Arsenal,Aaronson Terminal"
+ },
+ {
+ "name": "Vesuvivis",
+ "pos_x": 44.375,
+ "pos_y": -91.875,
+ "pos_z": 153.53125,
+ "stations": "Conway Landing"
+ },
+ {
+ "name": "Vetr",
+ "pos_x": -59.0625,
+ "pos_y": 12.65625,
+ "pos_z": 1.46875,
+ "stations": "Ehrlich City,Aristotle Horizons,Fossey Silo"
+ },
+ {
+ "name": "Vetta",
+ "pos_x": -43.625,
+ "pos_y": -46.5,
+ "pos_z": -34.46875,
+ "stations": "Bobko Terminal,Belyayev Dock,Langsdorff Holdings"
+ },
+ {
+ "name": "Vettones",
+ "pos_x": 7.4375,
+ "pos_y": -113.09375,
+ "pos_z": -98.125,
+ "stations": "McIntyre City,Pickering Point"
+ },
+ {
+ "name": "Vettorasare",
+ "pos_x": 129.40625,
+ "pos_y": -260,
+ "pos_z": -17.03125,
+ "stations": "Fan Outpost"
+ },
+ {
+ "name": "Vibio",
+ "pos_x": 116.21875,
+ "pos_y": 34.8125,
+ "pos_z": -95.0625,
+ "stations": "Zhen Depot,Harrison Installation"
+ },
+ {
+ "name": "Vidattuns",
+ "pos_x": -55.21875,
+ "pos_y": -66.21875,
+ "pos_z": 33.34375,
+ "stations": "Reightler Colony"
+ },
+ {
+ "name": "Vidavanta",
+ "pos_x": -50.9375,
+ "pos_y": 90.21875,
+ "pos_z": 65.8125,
+ "stations": "Lee Mines"
+ },
+ {
+ "name": "Vidu",
+ "pos_x": 132.75,
+ "pos_y": -31.15625,
+ "pos_z": 3.6875,
+ "stations": "Lyulka Plant,Bok Estate,Rodrigues Arsenal"
+ },
+ {
+ "name": "Viducasses",
+ "pos_x": -54.3125,
+ "pos_y": 78.5,
+ "pos_z": -72.125,
+ "stations": "Newton Depot"
+ },
+ {
+ "name": "Vigr",
+ "pos_x": -79.09375,
+ "pos_y": -39.875,
+ "pos_z": -28.25,
+ "stations": "McMonagle Landing"
+ },
+ {
+ "name": "Vili",
+ "pos_x": -11.03125,
+ "pos_y": -114.09375,
+ "pos_z": 34.875,
+ "stations": "Lintott's Inheritance"
+ },
+ {
+ "name": "Vilia",
+ "pos_x": 161.5625,
+ "pos_y": 37.53125,
+ "pos_z": 56.5,
+ "stations": "Birmingham Legacy"
+ },
+ {
+ "name": "Viliquerret",
+ "pos_x": -102.4375,
+ "pos_y": -95.53125,
+ "pos_z": 6.875,
+ "stations": "Cousin Hub"
+ },
+ {
+ "name": "Villae",
+ "pos_x": 101.25,
+ "pos_y": -192.375,
+ "pos_z": 93.15625,
+ "stations": "Drake Colony,Wood Vision,Mouhot Terminal,Burckhardt Landing"
+ },
+ {
+ "name": "Villeachi",
+ "pos_x": -19.09375,
+ "pos_y": -63.125,
+ "pos_z": 89.40625,
+ "stations": "Aksyonov Terminal,Worden Depot,Alvares Horizons"
+ },
+ {
+ "name": "Villing",
+ "pos_x": 69.65625,
+ "pos_y": -135.6875,
+ "pos_z": -109.1875,
+ "stations": "Daley Mines,Smith Reach"
+ },
+ {
+ "name": "Vimur",
+ "pos_x": 16.78125,
+ "pos_y": -4.125,
+ "pos_z": 101.53125,
+ "stations": "Ricardo Terminal,Baturin Port,Ali Station,Hausdorff Plant"
+ },
+ {
+ "name": "Vinab",
+ "pos_x": 42.21875,
+ "pos_y": -7.34375,
+ "pos_z": 137.6875,
+ "stations": "Caselberg Settlement,Barr Plant"
+ },
+ {
+ "name": "Vinansans",
+ "pos_x": 131.46875,
+ "pos_y": -120.125,
+ "pos_z": -73.1875,
+ "stations": "Stackpole Arena,Zander Landing"
+ },
+ {
+ "name": "Vincana",
+ "pos_x": 48.1875,
+ "pos_y": -132.9375,
+ "pos_z": 167.375,
+ "stations": "Freas Survey,Taine Point"
+ },
+ {
+ "name": "Vinciani",
+ "pos_x": 148.25,
+ "pos_y": -132.4375,
+ "pos_z": 1.34375,
+ "stations": "Adragna Station,Lundmark Ring,Ikeya Hub,Hoerner Orbital,Lee Prospect,South's Inheritance,Herodotus Silo,Cassini Hub,Wigura Port,Jones Orbital,Mil Port,Agrippa Port,Raleigh Settlement,Hamilton Relay"
+ },
+ {
+ "name": "Vincika",
+ "pos_x": 37.6875,
+ "pos_y": 137.90625,
+ "pos_z": -44.96875,
+ "stations": "Searfoss Horizons,Meucci Horizons,Forest's Progress"
+ },
+ {
+ "name": "Vindinepu",
+ "pos_x": 52.96875,
+ "pos_y": -3.65625,
+ "pos_z": 99.25,
+ "stations": "Shatalov Terminal,Coulter Base,Gamow Port"
+ },
+ {
+ "name": "Vindumbiva",
+ "pos_x": -78.3125,
+ "pos_y": -52.34375,
+ "pos_z": 122.71875,
+ "stations": "Polyakov's Claim"
+ },
+ {
+ "name": "Viracocha",
+ "pos_x": 14,
+ "pos_y": -46.4375,
+ "pos_z": 9.65625,
+ "stations": "Fife Legacy,Outpost Clarkie,Pataarcy Corporate"
+ },
+ {
+ "name": "Virangler",
+ "pos_x": 142.78125,
+ "pos_y": -133.25,
+ "pos_z": 124.6875,
+ "stations": "Reinhold's Progress"
+ },
+ {
+ "name": "Virawn",
+ "pos_x": 99.125,
+ "pos_y": 55.09375,
+ "pos_z": 40.28125,
+ "stations": "Horch Terminal"
+ },
+ {
+ "name": "Viro",
+ "pos_x": 97.90625,
+ "pos_y": -147.625,
+ "pos_z": -75.1875,
+ "stations": "Henderson Station,Rosenberger Vision,Palitzsch Gateway"
+ },
+ {
+ "name": "Viroluk",
+ "pos_x": -17.96875,
+ "pos_y": 100.5,
+ "pos_z": 0.96875,
+ "stations": "Levy Vision"
+ },
+ {
+ "name": "Viroman",
+ "pos_x": 119.6875,
+ "pos_y": -104.34375,
+ "pos_z": 74.21875,
+ "stations": "Tully Prospect,Goryu Prospect,Potocnik Vision"
+ },
+ {
+ "name": "Viromi",
+ "pos_x": 142.46875,
+ "pos_y": -171.53125,
+ "pos_z": -60.90625,
+ "stations": "Walotsky Orbital,Hartog Vision,Yakovlev Port,Hay Port,Liouville Settlement"
+ },
+ {
+ "name": "Viromovoi",
+ "pos_x": -19.90625,
+ "pos_y": 57.90625,
+ "pos_z": 130,
+ "stations": "Haxel Platform,Brooks' Inheritance"
+ },
+ {
+ "name": "Viron",
+ "pos_x": 161.6875,
+ "pos_y": -179.96875,
+ "pos_z": -57.46875,
+ "stations": "Narlikar Landing,Efremov Relay"
+ },
+ {
+ "name": "Virong",
+ "pos_x": 95.75,
+ "pos_y": -198.75,
+ "pos_z": -43,
+ "stations": "Kirkwood Terminal,Tombaugh Landing,Holub Prospect"
+ },
+ {
+ "name": "Virseku",
+ "pos_x": -130.34375,
+ "pos_y": -97.9375,
+ "pos_z": -35.6875,
+ "stations": "Hauck Platform"
+ },
+ {
+ "name": "Virset",
+ "pos_x": -75.125,
+ "pos_y": 112.59375,
+ "pos_z": 45.09375,
+ "stations": "Underwood Terminal"
+ },
+ {
+ "name": "Viruachi",
+ "pos_x": -82.84375,
+ "pos_y": 79.03125,
+ "pos_z": -23.09375,
+ "stations": "Alexander Port,Cortes Prospect,Koontz Installation"
+ },
+ {
+ "name": "Virud",
+ "pos_x": -60.75,
+ "pos_y": -9.6875,
+ "pos_z": 81.625,
+ "stations": "Beadle Hangar"
+ },
+ {
+ "name": "Virudnir",
+ "pos_x": -12.78125,
+ "pos_y": 143.28125,
+ "pos_z": -66.125,
+ "stations": "Rennie Dock"
+ },
+ {
+ "name": "Virung",
+ "pos_x": 0,
+ "pos_y": 19.40625,
+ "pos_z": -164.09375,
+ "stations": "Massimino Station,Bluford Hub,McArthur Dock,Garratt Installation,Ray Holdings"
+ },
+ {
+ "name": "Virus",
+ "pos_x": -43.03125,
+ "pos_y": -34.4375,
+ "pos_z": 117.5625,
+ "stations": "Macgregor Dock,Stuart Installation,Dirichlet Landing,Grothendieck Installation"
+ },
+ {
+ "name": "Virusalansi",
+ "pos_x": 90.28125,
+ "pos_y": -110.96875,
+ "pos_z": -121.15625,
+ "stations": "VanderMeer Enterprise,Bass Ring,Williamson Mines,Yano Installation,Klink Installation"
+ },
+ {
+ "name": "Vish",
+ "pos_x": 134.40625,
+ "pos_y": -136.25,
+ "pos_z": -16.40625,
+ "stations": "Ryle Vision,d'Arrest Orbital,Clark Base,Austin Horizons"
+ },
+ {
+ "name": "Vishepeu",
+ "pos_x": 105.03125,
+ "pos_y": -4.5,
+ "pos_z": -111.21875,
+ "stations": "Ray Platform,Eckford Settlement"
+ },
+ {
+ "name": "Vishi",
+ "pos_x": 51.09375,
+ "pos_y": 12.875,
+ "pos_z": -157.09375,
+ "stations": "Jolliet Colony,Keyes Survey,Grant Arsenal"
+ },
+ {
+ "name": "Vishkyamu",
+ "pos_x": -134.0625,
+ "pos_y": -47.875,
+ "pos_z": 48.625,
+ "stations": "Dozois Mine,Kagan Escape"
+ },
+ {
+ "name": "Vishnaya",
+ "pos_x": -40.90625,
+ "pos_y": -115.375,
+ "pos_z": 114,
+ "stations": "Brorsen Horizons,Faber Dock,Schmidt Installation,Zajdel Laboratory"
+ },
+ {
+ "name": "Visho",
+ "pos_x": 40.4375,
+ "pos_y": -216.625,
+ "pos_z": 23.5,
+ "stations": "Rich Orbital,Gora Terminal,Oshima Terminal,Sucharitkul Prospect,O'Leary Lab"
+ },
+ {
+ "name": "Vishtha",
+ "pos_x": -36,
+ "pos_y": 25.03125,
+ "pos_z": 124.5625,
+ "stations": "Vishweswarayya Station,Kizim Port,Furukawa Gateway"
+ },
+ {
+ "name": "Vishvamitra",
+ "pos_x": 63.6875,
+ "pos_y": -98.28125,
+ "pos_z": 120.625,
+ "stations": "Williams Settlement,Mayer Enterprise,Haack Station,Hiyya City,Bixby's Progress,Flammarion Terminal"
+ },
+ {
+ "name": "Vishvins",
+ "pos_x": -3.3125,
+ "pos_y": -12.71875,
+ "pos_z": 138.84375,
+ "stations": "Alexeyev Port"
+ },
+ {
+ "name": "Vistaenis",
+ "pos_x": 102.5,
+ "pos_y": -104.78125,
+ "pos_z": 28.65625,
+ "stations": "Youll Port,Meinel Penal colony,Naburimannu Ring,Merritt Landing,Uto Port,Samos Terminal"
+ },
+ {
+ "name": "Visthalam",
+ "pos_x": 159.5,
+ "pos_y": -107.46875,
+ "pos_z": 40.6875,
+ "stations": "van der Riet Woolley Ring,Ball Horizons,Lyot Beacon"
+ },
+ {
+ "name": "Vistnero",
+ "pos_x": -142.71875,
+ "pos_y": -42.96875,
+ "pos_z": -35.5,
+ "stations": "Drexler City,Harris Terminal,Torricelli Hub,Laval Terminal"
+ },
+ {
+ "name": "Visto",
+ "pos_x": 125.09375,
+ "pos_y": -7.625,
+ "pos_z": 78.8125,
+ "stations": "Makeev Bastion,Macarthur Works,Jemison Dock"
+ },
+ {
+ "name": "Vistobici",
+ "pos_x": -24.71875,
+ "pos_y": -23.78125,
+ "pos_z": 102.5,
+ "stations": "Linaweaver Hub,Rey Camp"
+ },
+ {
+ "name": "Vistsi",
+ "pos_x": 95.65625,
+ "pos_y": 37.6875,
+ "pos_z": -83.84375,
+ "stations": "Aleksandrov Colony,Faris Settlement"
+ },
+ {
+ "name": "Vito",
+ "pos_x": 153.5,
+ "pos_y": 72.09375,
+ "pos_z": -43.03125,
+ "stations": "Stokes' Folly,Farmer Relay,Hilbert Stop"
+ },
+ {
+ "name": "Vitoa",
+ "pos_x": -0.71875,
+ "pos_y": 20.78125,
+ "pos_z": 84.53125,
+ "stations": "Mitchell Dock,Bobko City,Galilei Station"
+ },
+ {
+ "name": "Vitola",
+ "pos_x": 131.4375,
+ "pos_y": -204.78125,
+ "pos_z": 0.375,
+ "stations": "van de Hulst Hub,Wolf Mines,Dunyach Vision"
+ },
+ {
+ "name": "Vitra",
+ "pos_x": 30.59375,
+ "pos_y": -116.46875,
+ "pos_z": -90.53125,
+ "stations": "Jahn Station,Bolotov Station,Linteris Dock,Powell Point"
+ },
+ {
+ "name": "Vitri",
+ "pos_x": -20.375,
+ "pos_y": -5.78125,
+ "pos_z": -171.5625,
+ "stations": "Vasilyev Enterprise,Anthony Barracks,Burton Station,Potagos Station"
+ },
+ {
+ "name": "Vivas",
+ "pos_x": -143.03125,
+ "pos_y": 37.4375,
+ "pos_z": 0.46875,
+ "stations": "Vercors Outpost"
+ },
+ {
+ "name": "Vivata",
+ "pos_x": 17.96875,
+ "pos_y": -77.375,
+ "pos_z": -17.59375,
+ "stations": "Oluwafemi Settlement"
+ },
+ {
+ "name": "Vivates",
+ "pos_x": 2.375,
+ "pos_y": -132.96875,
+ "pos_z": -62.625,
+ "stations": "Schwarzschild Platform,von Zach Vision,Hawker Depot"
+ },
+ {
+ "name": "Vocorimpus",
+ "pos_x": 78.09375,
+ "pos_y": -221.0625,
+ "pos_z": 118.75,
+ "stations": "Jackson Relay"
+ },
+ {
+ "name": "Vocorimur",
+ "pos_x": 135.375,
+ "pos_y": 58.125,
+ "pos_z": 78.65625,
+ "stations": "Robinson Prospect,Ing Beacon"
+ },
+ {
+ "name": "Vocovii",
+ "pos_x": 138.75,
+ "pos_y": 28.59375,
+ "pos_z": -109.46875,
+ "stations": "Kornbluth Point,Eckford Oasis,Clayton Settlement"
+ },
+ {
+ "name": "Vodnirtama",
+ "pos_x": -118.25,
+ "pos_y": -21.65625,
+ "pos_z": -61.65625,
+ "stations": "Crumey Mines,Perez's Claim,Flint Refinery"
+ },
+ {
+ "name": "Vodyak",
+ "pos_x": -27.3125,
+ "pos_y": 140.90625,
+ "pos_z": 40.875,
+ "stations": "Markham Legacy"
+ },
+ {
+ "name": "Vodyakamana",
+ "pos_x": -115.25,
+ "pos_y": 132.8125,
+ "pos_z": 15.5625,
+ "stations": "Schade Vision,Shepard Landing,Stanley Depot"
+ },
+ {
+ "name": "Vodyanes",
+ "pos_x": 80.21875,
+ "pos_y": -55.65625,
+ "pos_z": -14.46875,
+ "stations": "Denning Vision,Dassault Orbital,Baynes Hub,Lewis Gateway,Drake Gateway,Bolton Enterprise,Kosai Terminal,Common Dock,Ernst Ring"
+ },
+ {
+ "name": "Vodyang",
+ "pos_x": 55.59375,
+ "pos_y": -34.25,
+ "pos_z": 135.375,
+ "stations": "Wylie Mine"
+ },
+ {
+ "name": "Vodyanoa",
+ "pos_x": 26.09375,
+ "pos_y": -213.65625,
+ "pos_z": 18.875,
+ "stations": "Struve Prospect,Kibalchich Keep"
+ },
+ {
+ "name": "Vogul",
+ "pos_x": 148.125,
+ "pos_y": -146.8125,
+ "pos_z": -34.5,
+ "stations": "Zhukovsky Terminal,Kondo Dock,Aller Terminal,Wagner Terminal"
+ },
+ {
+ "name": "Vogulmangoi",
+ "pos_x": 94.40625,
+ "pos_y": -48.96875,
+ "pos_z": -128.75,
+ "stations": "Crick Outpost,Knipling Point"
+ },
+ {
+ "name": "Vogulu",
+ "pos_x": 159.84375,
+ "pos_y": -89.8125,
+ "pos_z": -45.59375,
+ "stations": "Finlay Hub,Steiner's Progress,Rizvi Terminal,Zhu Survey"
+ },
+ {
+ "name": "Voguluan",
+ "pos_x": 126.21875,
+ "pos_y": 3.5625,
+ "pos_z": 41.46875,
+ "stations": "Born Relay,Serrao Dock,Schmitz Terminal"
+ },
+ {
+ "name": "Volcadra",
+ "pos_x": -25,
+ "pos_y": -156.84375,
+ "pos_z": 27.15625,
+ "stations": "Hussey Platform,Frazetta Hub"
+ },
+ {
+ "name": "Volcae",
+ "pos_x": -120,
+ "pos_y": -20.15625,
+ "pos_z": -75.34375,
+ "stations": "Holden Ring,Roggeveen Survey,Delany Horizons"
+ },
+ {
+ "name": "Volcani",
+ "pos_x": 155.6875,
+ "pos_y": -123.09375,
+ "pos_z": 55.5,
+ "stations": "Barnard Station,Hirn Depot,McManus Hub,Gunter City"
+ },
+ {
+ "name": "Volcay",
+ "pos_x": -7.5625,
+ "pos_y": -200.03125,
+ "pos_z": 103.40625,
+ "stations": "Husband Landing,Dyr Prospect"
+ },
+ {
+ "name": "Volcayayp",
+ "pos_x": 23.28125,
+ "pos_y": -62.125,
+ "pos_z": 156.0625,
+ "stations": "Tolstoi Platform,Priest Point"
+ },
+ {
+ "name": "Volciani",
+ "pos_x": 120.875,
+ "pos_y": -180.15625,
+ "pos_z": 11.09375,
+ "stations": "Messerschmitt Settlement"
+ },
+ {
+ "name": "Volciates",
+ "pos_x": -126.625,
+ "pos_y": 7.8125,
+ "pos_z": 74.6875,
+ "stations": "Hopkins Terminal,Bobko Orbital,Nasmyth Landing,Dirac Port,Bertin Holdings"
+ },
+ {
+ "name": "Volhynians",
+ "pos_x": 26.46875,
+ "pos_y": -45.40625,
+ "pos_z": -73.03125,
+ "stations": "Liebig Beacon"
+ },
+ {
+ "name": "Volithigga",
+ "pos_x": -80.46875,
+ "pos_y": -64.5,
+ "pos_z": -46.96875,
+ "stations": "Still Dock,Tarentum Point"
+ },
+ {
+ "name": "Volkhab",
+ "pos_x": 36.53125,
+ "pos_y": 100.0625,
+ "pos_z": -135.71875,
+ "stations": "Vernadsky Dock,Schweickart Hub,Gutierrez Terminal,Lindbohm Keep"
+ },
+ {
+ "name": "Volkhabe",
+ "pos_x": -56.90625,
+ "pos_y": -75.1875,
+ "pos_z": -76.84375,
+ "stations": "Cook Arsenal,Offutt Enterprise,Galiano Station,Leichhardt Landing,Alvarez de Pineda Terminal"
+ },
+ {
+ "name": "Volo",
+ "pos_x": 121.625,
+ "pos_y": 69.4375,
+ "pos_z": -88.0625,
+ "stations": "Ponce de Leon Retreat"
+ },
+ {
+ "name": "Volowahku",
+ "pos_x": 128.65625,
+ "pos_y": 95.25,
+ "pos_z": 78.65625,
+ "stations": "Lee Terminal,Watt Mine"
+ },
+ {
+ "name": "Volscii",
+ "pos_x": 97.0625,
+ "pos_y": -180.4375,
+ "pos_z": 139.90625,
+ "stations": "Sternbach Colony"
+ },
+ {
+ "name": "Volsum",
+ "pos_x": 73.65625,
+ "pos_y": -15.15625,
+ "pos_z": 158.78125,
+ "stations": "Longyear Terminal,Cleve Hub"
+ },
+ {
+ "name": "Voltani",
+ "pos_x": -8.90625,
+ "pos_y": -136.03125,
+ "pos_z": 57.34375,
+ "stations": "Shen Camp,Oren Vision"
+ },
+ {
+ "name": "Voltrigones",
+ "pos_x": 96.0625,
+ "pos_y": -11.28125,
+ "pos_z": 104.375,
+ "stations": "Smith Colony,Marley Barracks,Low Dock"
+ },
+ {
+ "name": "Volu",
+ "pos_x": 76.75,
+ "pos_y": -5.15625,
+ "pos_z": -62.59375,
+ "stations": "Popovich Landing"
+ },
+ {
+ "name": "Volungu",
+ "pos_x": -25.5,
+ "pos_y": -10.5,
+ "pos_z": 36.96875,
+ "stations": "Jemison Refinery,Cabrera Horizons,Hogan Point"
+ },
+ {
+ "name": "Volupana",
+ "pos_x": 156.03125,
+ "pos_y": -200.6875,
+ "pos_z": -1.5,
+ "stations": "Vercors Prospect,McManus Survey,Auwers Platform"
+ },
+ {
+ "name": "Volupanu",
+ "pos_x": 108.46875,
+ "pos_y": -102.8125,
+ "pos_z": 132.5625,
+ "stations": "Whelan Vision,Wilhelm Mines"
+ },
+ {
+ "name": "Vorden",
+ "pos_x": -170.28125,
+ "pos_y": 1.28125,
+ "pos_z": -19.71875,
+ "stations": "Borel Dock,Cremona Dock,Moresby Laboratory,Rothman Installation"
+ },
+ {
+ "name": "Vordengha",
+ "pos_x": -24.3125,
+ "pos_y": -23.125,
+ "pos_z": 100.875,
+ "stations": "Zhigang Mine,Lockhart Settlement,Edgeworth Hub"
+ },
+ {
+ "name": "Vordini",
+ "pos_x": 157.125,
+ "pos_y": -67.625,
+ "pos_z": 23,
+ "stations": "Valigursky Survey,Bickel Terminal,Barnaby Depot"
+ },
+ {
+ "name": "Vorduli",
+ "pos_x": 116.1875,
+ "pos_y": 4.1875,
+ "pos_z": -141.25,
+ "stations": "Bao City,Preuss Port"
+ },
+ {
+ "name": "Vota",
+ "pos_x": 106.875,
+ "pos_y": -73.375,
+ "pos_z": -85.78125,
+ "stations": "Pittendreigh Gateway,Sopwith Port,Zhukovsky Vision,Harvey-Smith Depot,Sitter Base,Holland Depot"
+ },
+ {
+ "name": "Votadini",
+ "pos_x": 165.53125,
+ "pos_y": -51.9375,
+ "pos_z": 24.34375,
+ "stations": "Chertovsky Vision"
+ },
+ {
+ "name": "Votadja",
+ "pos_x": 165.6875,
+ "pos_y": -184.90625,
+ "pos_z": 90.28125,
+ "stations": "Royo Hub,Gilliland Base,Chiang Landing"
+ },
+ {
+ "name": "Votai",
+ "pos_x": -107.96875,
+ "pos_y": 7.125,
+ "pos_z": 28.6875,
+ "stations": "Buckey Platform,Teller Terminal,Tognini Port,Kessel Depot"
+ },
+ {
+ "name": "Votama",
+ "pos_x": 93.8125,
+ "pos_y": -14.9375,
+ "pos_z": 12.15625,
+ "stations": "Tarentum Mine,Van Scyoc Survey,Williams Platform,Clark Arsenal"
+ },
+ {
+ "name": "Votaroi",
+ "pos_x": 97.84375,
+ "pos_y": -77.09375,
+ "pos_z": -42,
+ "stations": "Hirayama Terminal,Baillaud Mines,Shinjo Vision,Molchanov's Inheritance"
+ },
+ {
+ "name": "Votat",
+ "pos_x": -53.75,
+ "pos_y": 69.6875,
+ "pos_z": 67.96875,
+ "stations": "Oluwafemi Vision,Aristotle's Progress"
+ },
+ {
+ "name": "Votatini",
+ "pos_x": -7.34375,
+ "pos_y": -123.875,
+ "pos_z": 8.71875,
+ "stations": "Schmidt Dock,King Terminal,Boyer Orbital,Valigursky Orbital,Wolf Installation,Lawhead Base"
+ },
+ {
+ "name": "Vucab Cakix",
+ "pos_x": 185.78125,
+ "pos_y": -134.03125,
+ "pos_z": 84.46875,
+ "stations": "Riebe Reach"
+ },
+ {
+ "name": "Vucainigus",
+ "pos_x": -84.09375,
+ "pos_y": 97.9375,
+ "pos_z": 17.625,
+ "stations": "Leavitt Port,Berezovoy Station"
+ },
+ {
+ "name": "Vucairu",
+ "pos_x": 9.15625,
+ "pos_y": 124.3125,
+ "pos_z": 7.375,
+ "stations": "Bottego Station,Clairaut Colony"
+ },
+ {
+ "name": "Vucasset",
+ "pos_x": -83.71875,
+ "pos_y": -67.4375,
+ "pos_z": -124.3125,
+ "stations": "Quinn Platform,Moore Retreat,Carter Hub"
+ },
+ {
+ "name": "Vucasto",
+ "pos_x": 26.5,
+ "pos_y": -130.34375,
+ "pos_z": -84.25,
+ "stations": "Struzan Gateway,Bingzhen Port,Arago Terminal,Wolf Depot"
+ },
+ {
+ "name": "Vucay",
+ "pos_x": 167.78125,
+ "pos_y": -167.03125,
+ "pos_z": -2.25,
+ "stations": "Airy Works"
+ },
+ {
+ "name": "Vucub Huan",
+ "pos_x": 65.3125,
+ "pos_y": 35.03125,
+ "pos_z": 52.25,
+ "stations": "Conrad Base,Walter Prospect"
+ },
+ {
+ "name": "Vucumanada",
+ "pos_x": 23.0625,
+ "pos_y": -153.21875,
+ "pos_z": 39.28125,
+ "stations": "Rechtin Dock"
+ },
+ {
+ "name": "Vucumatha",
+ "pos_x": 137.84375,
+ "pos_y": 25.875,
+ "pos_z": -97.8125,
+ "stations": "Champlain Orbital,Erikson Horizons"
+ },
+ {
+ "name": "Vucurici",
+ "pos_x": 14.875,
+ "pos_y": -99.8125,
+ "pos_z": -75.90625,
+ "stations": "Macquorn Rankine Works,Bernoulli Terminal"
+ },
+ {
+ "name": "Vucurun",
+ "pos_x": -87.0625,
+ "pos_y": 87.25,
+ "pos_z": -87.96875,
+ "stations": "Reed Vision,Barcelos Holdings,Clairaut Colony,Besonders Base"
+ },
+ {
+ "name": "Vukuapa",
+ "pos_x": -63.875,
+ "pos_y": 64.84375,
+ "pos_z": -47.25,
+ "stations": "Mendeleev Terminal"
+ },
+ {
+ "name": "Vukun",
+ "pos_x": -114.3125,
+ "pos_y": -44.40625,
+ "pos_z": 6.84375,
+ "stations": "Selberg Reach"
+ },
+ {
+ "name": "Vukuna",
+ "pos_x": -45.15625,
+ "pos_y": 50.1875,
+ "pos_z": 101.4375,
+ "stations": "Bagian Ring"
+ },
+ {
+ "name": "Vukur",
+ "pos_x": -15.96875,
+ "pos_y": -14.46875,
+ "pos_z": -105.65625,
+ "stations": "Armstrong Settlement,Bohr Refinery"
+ },
+ {
+ "name": "Vukuracians",
+ "pos_x": 88.34375,
+ "pos_y": -143.96875,
+ "pos_z": 115.90625,
+ "stations": "Herbig Station,Blair Works,Borrego Station,Hoyle's Claim,Beaumont's Folly,Bouvard Station"
+ },
+ {
+ "name": "Vukurbeh",
+ "pos_x": 87.0625,
+ "pos_y": -70.03125,
+ "pos_z": -98.28125,
+ "stations": "Bernoulli Landing,Mitra Hub,Bishop Enterprise,Gardner Vision"
+ },
+ {
+ "name": "Vukurnir",
+ "pos_x": 114.03125,
+ "pos_y": 19.90625,
+ "pos_z": -25.65625,
+ "stations": "Zudov Relay,Hartlib Holdings,Phillips Orbital"
+ },
+ {
+ "name": "Vuldr",
+ "pos_x": -28.8125,
+ "pos_y": -96.59375,
+ "pos_z": 62.59375,
+ "stations": "McBride Port,Gibson Orbital,Rushd Camp"
+ },
+ {
+ "name": "Vuldrajo",
+ "pos_x": -33.3125,
+ "pos_y": 60.125,
+ "pos_z": 100.625,
+ "stations": "Padalka Terminal,Born City,Sharma Terminal,Shepard Installation,Ahmed Point"
+ },
+ {
+ "name": "Vulganji",
+ "pos_x": 117.875,
+ "pos_y": -12.5625,
+ "pos_z": -73.0625,
+ "stations": "Erikson Port"
+ },
+ {
+ "name": "VVO 19",
+ "pos_x": -42.03125,
+ "pos_y": -46.15625,
+ "pos_z": 46.21875,
+ "stations": "Leavitt Port,Viktorenko Station,Harper Base,Hawking's Folly,Kaku Station,Bohr Terminal,Meade Port"
+ },
+ {
+ "name": "Vyat",
+ "pos_x": 96.28125,
+ "pos_y": 20.09375,
+ "pos_z": -31.4375,
+ "stations": "Blackman Sanctuary,Herodotus Prospect,Morrow Terminal"
+ },
+ {
+ "name": "Vyatsyapod",
+ "pos_x": -122.5625,
+ "pos_y": -32.46875,
+ "pos_z": 7.09375,
+ "stations": "Morey Vision,Tognini Depot"
+ },
+ {
+ "name": "VZ Columbae",
+ "pos_x": 48.15625,
+ "pos_y": -32.59375,
+ "pos_z": -19.28125,
+ "stations": "Pogue Barracks,Liouville Landing,Sleator City"
+ },
+ {
+ "name": "VZ Corvi",
+ "pos_x": 39.6875,
+ "pos_y": 50.4375,
+ "pos_z": 25.59375,
+ "stations": "Neville Ring,Steakley Base,Comer Terminal,Malcolm Ring,Archer Station,Bear Settlement,Davidson Station,Pullman Terminal,Ashton Gateway,Hoshi Enterprise,Harding Ring,Waldrop Enterprise"
+ },
+ {
+ "name": "W Ursae Majoris",
+ "pos_x": -40,
+ "pos_y": 117.15625,
+ "pos_z": -104.03125,
+ "stations": "Asher Vista"
+ },
+ {
+ "name": "Waang",
+ "pos_x": -112,
+ "pos_y": -75.9375,
+ "pos_z": -96.625,
+ "stations": "Foreman Point"
+ },
+ {
+ "name": "Waangamici",
+ "pos_x": 18.65625,
+ "pos_y": -136.21875,
+ "pos_z": 1.5,
+ "stations": "Kovalevskaya Outpost"
+ },
+ {
+ "name": "Waba",
+ "pos_x": -12.28125,
+ "pos_y": -119.03125,
+ "pos_z": 15.59375,
+ "stations": "Korlevic Station,Baade Installation,William Sargent Hub,Rolland Exchange"
+ },
+ {
+ "name": "Wababa",
+ "pos_x": 139.1875,
+ "pos_y": -167.625,
+ "pos_z": 99.46875,
+ "stations": "Michell City,Roelofs Station,Maupertuis Orbital,Ricci Base"
+ },
+ {
+ "name": "Wababaye",
+ "pos_x": 116.6875,
+ "pos_y": -41.53125,
+ "pos_z": 46.5625,
+ "stations": "Naylor City,Auer Point"
+ },
+ {
+ "name": "Wabayo",
+ "pos_x": 123.46875,
+ "pos_y": -88.21875,
+ "pos_z": 43.34375,
+ "stations": "Hardy Orbital,Thorne Ring"
+ },
+ {
+ "name": "Wader",
+ "pos_x": 115.71875,
+ "pos_y": 89.78125,
+ "pos_z": 89.875,
+ "stations": "Williams Hub,Sharp Vision,Elcano Installation"
+ },
+ {
+ "name": "Wadillae",
+ "pos_x": -129.9375,
+ "pos_y": -2.0625,
+ "pos_z": -12.125,
+ "stations": "Dunn Arsenal,Dedekind Beacon,Conti Refinery"
+ },
+ {
+ "name": "Wadimurt",
+ "pos_x": 156.0625,
+ "pos_y": -126.78125,
+ "pos_z": 96.15625,
+ "stations": "Furukawa Port,Hampson Hub,Keyes' Progress"
+ },
+ {
+ "name": "Wadir",
+ "pos_x": -42.9375,
+ "pos_y": -66.6875,
+ "pos_z": 139.84375,
+ "stations": "Hoften Port,Harris Installation,Detmer Dock"
+ },
+ {
+ "name": "Wadj Wen",
+ "pos_x": 54.8125,
+ "pos_y": -173.21875,
+ "pos_z": 106.375,
+ "stations": "Fawcett Prospect,Faye's Claim"
+ },
+ {
+ "name": "Wadjabangai",
+ "pos_x": -24.53125,
+ "pos_y": -33.78125,
+ "pos_z": 108.8125,
+ "stations": "Gromov Horizons"
+ },
+ {
+ "name": "Wadjalang",
+ "pos_x": 158.375,
+ "pos_y": -42.6875,
+ "pos_z": -7.5625,
+ "stations": "Dolgov Port"
+ },
+ {
+ "name": "Wadjali",
+ "pos_x": -34.8125,
+ "pos_y": -42.84375,
+ "pos_z": 70.875,
+ "stations": "Trevithick Terminal,White Station,Kidman Terminal,Siemens Orbital,Great Installation,Herreshoff Keep,Meikle Port,Vetulani Port,Tarelkin Port"
+ },
+ {
+ "name": "Wadjalkan",
+ "pos_x": -94.09375,
+ "pos_y": -63.375,
+ "pos_z": 19.96875,
+ "stations": "Potter Station,Maclaurin Installation"
+ },
+ {
+ "name": "Wadjambaru",
+ "pos_x": -109.21875,
+ "pos_y": -3.25,
+ "pos_z": 45.6875,
+ "stations": "Kavandi Landing"
+ },
+ {
+ "name": "Wadjang",
+ "pos_x": -55.1875,
+ "pos_y": -103.1875,
+ "pos_z": -95.4375,
+ "stations": "Whitney Landing"
+ },
+ {
+ "name": "Wadjarata",
+ "pos_x": -69.65625,
+ "pos_y": -78.40625,
+ "pos_z": 48.75,
+ "stations": "Rosenberg Hub,Salak Base,Greenleaf Refinery"
+ },
+ {
+ "name": "Wadjari",
+ "pos_x": 7.15625,
+ "pos_y": -102.34375,
+ "pos_z": -85.375,
+ "stations": "Borlaug Orbital"
+ },
+ {
+ "name": "Wadjarimpo",
+ "pos_x": -90.3125,
+ "pos_y": -31.21875,
+ "pos_z": 23.34375,
+ "stations": "Stephenson Orbital"
+ },
+ {
+ "name": "Wadjedet",
+ "pos_x": 203.53125,
+ "pos_y": -108.78125,
+ "pos_z": 0.21875,
+ "stations": "Unsold Orbital,Palisa Vision,Bus Gateway,Akiyama Beacon,Walters Point"
+ },
+ {
+ "name": "Wadjetese",
+ "pos_x": -149.75,
+ "pos_y": 31.96875,
+ "pos_z": 94.21875,
+ "stations": "Parazynski Outpost,Kuhn Enterprise"
+ },
+ {
+ "name": "Wadjey'mi",
+ "pos_x": -89.8125,
+ "pos_y": -93.15625,
+ "pos_z": -23.40625,
+ "stations": "Wrangel Horizons,Whitelaw Penal colony,Gibson Point"
+ },
+ {
+ "name": "Wadjimburr",
+ "pos_x": -13.0625,
+ "pos_y": 126.03125,
+ "pos_z": 82.8125,
+ "stations": "Neff Beacon"
+ },
+ {
+ "name": "Wadjirusi",
+ "pos_x": 100.5625,
+ "pos_y": -225.78125,
+ "pos_z": 77.375,
+ "stations": "Wegener Port,Goodricke Platform,Gould Horizons,J. G. Ballard Escape"
+ },
+ {
+ "name": "Wadjuk",
+ "pos_x": -50.59375,
+ "pos_y": 49.84375,
+ "pos_z": -45.8125,
+ "stations": "Lucretius Hub,Song Orbital,Bose Station,Haisheng Port,Cobb Laboratory,Hawley Terminal,Baudry Hub,Mills Laboratory,Zhigang Enterprise,Crown Barracks"
+ },
+ {
+ "name": "Wadjuna",
+ "pos_x": -19.28125,
+ "pos_y": -129.28125,
+ "pos_z": 86.125,
+ "stations": "Wilhelm von Struve Settlement,Williamson Base,Rankin Platform"
+ },
+ {
+ "name": "Waginyin",
+ "pos_x": -32.03125,
+ "pos_y": 103.6875,
+ "pos_z": -40.28125,
+ "stations": "Weber Orbital,Lerner Installation,Windt Vision"
+ },
+ {
+ "name": "Waikino",
+ "pos_x": -9.875,
+ "pos_y": 45.53125,
+ "pos_z": -140.9375,
+ "stations": "Kagan Mines,Zindell Enterprise,Merchiston Beacon"
+ },
+ {
+ "name": "Waikiri",
+ "pos_x": 92.75,
+ "pos_y": 136.5,
+ "pos_z": -23.90625,
+ "stations": "Robinson Colony,Baker Settlement,Burnham Plant"
+ },
+ {
+ "name": "Waikpoi",
+ "pos_x": 61.34375,
+ "pos_y": -50.09375,
+ "pos_z": 139.75,
+ "stations": "Friedman Relay"
+ },
+ {
+ "name": "Waikula",
+ "pos_x": -125.8125,
+ "pos_y": 31.90625,
+ "pos_z": 4.875,
+ "stations": "Wallace Port,Young Depot,Barba Port,Wang Landing"
+ },
+ {
+ "name": "Wail",
+ "pos_x": 104.5,
+ "pos_y": 124.28125,
+ "pos_z": 9.03125,
+ "stations": "Roberts Dock,Mitchison Terminal"
+ },
+ {
+ "name": "Waila",
+ "pos_x": -21.5625,
+ "pos_y": -202.15625,
+ "pos_z": -43.59375,
+ "stations": "Pacheco Outpost"
+ },
+ {
+ "name": "Wailaroju",
+ "pos_x": 50.625,
+ "pos_y": -47.21875,
+ "pos_z": -44.40625,
+ "stations": "Galvani Hub,Low Hub,Joliot-Curie Gateway,Boas Terminal,Nespoli Hub,Evangelisti Enterprise,Elder's Inheritance,Herzfeld Hub"
+ },
+ {
+ "name": "Wailla Sakh",
+ "pos_x": -19.03125,
+ "pos_y": -52.125,
+ "pos_z": 117.625,
+ "stations": "Harvey Orbital,Baker Port,Spring City,Alexandria's Inheritance,Garrett Bastion,Bushkov's Inheritance"
+ },
+ {
+ "name": "Wailladyan",
+ "pos_x": -22.71875,
+ "pos_y": -32.53125,
+ "pos_z": 40.71875,
+ "stations": "Bass Port"
+ },
+ {
+ "name": "Wailli",
+ "pos_x": 157.9375,
+ "pos_y": -63.21875,
+ "pos_z": 70.59375,
+ "stations": "Wnuk-Lipinski Settlement,Crown Station,Tepper Penal colony"
+ },
+ {
+ "name": "Waillingga",
+ "pos_x": 21.09375,
+ "pos_y": 115.125,
+ "pos_z": -39.5625,
+ "stations": "Yano City,Shiner Prospect"
+ },
+ {
+ "name": "Wailtsu",
+ "pos_x": -98.25,
+ "pos_y": -39.5,
+ "pos_z": 98,
+ "stations": "Stillman Survey,Barreiros Depot"
+ },
+ {
+ "name": "Waima",
+ "pos_x": 152.71875,
+ "pos_y": -33.78125,
+ "pos_z": 7.8125,
+ "stations": "Pudwill Gorie Hub,Pashin Vista,Sullivan Port"
+ },
+ {
+ "name": "Waime",
+ "pos_x": 141.53125,
+ "pos_y": -163.59375,
+ "pos_z": 107.6875,
+ "stations": "Nojiri Prospect,Heinemann Oasis"
+ },
+ {
+ "name": "Waimi",
+ "pos_x": -58.9375,
+ "pos_y": -116.90625,
+ "pos_z": -11.125,
+ "stations": "Hahn Dock,Tyson Orbital"
+ },
+ {
+ "name": "Waimile",
+ "pos_x": 128.5,
+ "pos_y": -202.40625,
+ "pos_z": 120.71875,
+ "stations": "Malvern Vision"
+ },
+ {
+ "name": "Waimiri",
+ "pos_x": 126,
+ "pos_y": -145.09375,
+ "pos_z": -42.5,
+ "stations": "Rich Ring,Foss Dock,Cummings Hub,Plancius' Progress"
+ },
+ {
+ "name": "Wak",
+ "pos_x": 14.0625,
+ "pos_y": 27.53125,
+ "pos_z": 105.21875,
+ "stations": "Lorrah City,Vlamingh Enterprise"
+ },
+ {
+ "name": "Waka Mu",
+ "pos_x": 11.15625,
+ "pos_y": 77.25,
+ "pos_z": 70.15625,
+ "stations": "Worlidge Camp,Aubakirov Mine,Kondakova Mines"
+ },
+ {
+ "name": "Wakait",
+ "pos_x": -30.15625,
+ "pos_y": 55.46875,
+ "pos_z": -119.1875,
+ "stations": "Gaspar de Lemos Ring,Pangborn Hub,Farouk Laboratory,Steakley Depot"
+ },
+ {
+ "name": "Wakakaduvul",
+ "pos_x": -52.25,
+ "pos_y": -2.5,
+ "pos_z": 128.75,
+ "stations": "Swanson Mines,Delany Vision,Slayton Landing"
+ },
+ {
+ "name": "Wakal",
+ "pos_x": -9.3125,
+ "pos_y": -18.59375,
+ "pos_z": 135.9375,
+ "stations": "Kelly Landing"
+ },
+ {
+ "name": "Wakam",
+ "pos_x": 88.09375,
+ "pos_y": -30.90625,
+ "pos_z": -94.59375,
+ "stations": "Avogadro Dock"
+ },
+ {
+ "name": "Wakandiini",
+ "pos_x": -32.09375,
+ "pos_y": -67.78125,
+ "pos_z": -130.25,
+ "stations": "Cooper Orbital,Szilard Hub,Crown Settlement"
+ },
+ {
+ "name": "Wakandji",
+ "pos_x": 85.6875,
+ "pos_y": 129.5,
+ "pos_z": 19.53125,
+ "stations": "Lucretius Orbital,Rzeppa Station,Manarov Enterprise,Reis Penal colony"
+ },
+ {
+ "name": "Wakans",
+ "pos_x": 97.28125,
+ "pos_y": 83.65625,
+ "pos_z": 38.875,
+ "stations": "Disch Relay,Regiomontanus Settlement,Ross Plant"
+ },
+ {
+ "name": "Wakaparibe",
+ "pos_x": 19.875,
+ "pos_y": -37.4375,
+ "pos_z": 3.65625,
+ "stations": "Lichtenberg Port"
+ },
+ {
+ "name": "Wakathime",
+ "pos_x": 114.75,
+ "pos_y": -203.0625,
+ "pos_z": -59.65625,
+ "stations": "Schwabe Depot"
+ },
+ {
+ "name": "Wakaulang",
+ "pos_x": -156.46875,
+ "pos_y": -31.15625,
+ "pos_z": 29.78125,
+ "stations": "Williams Depot,Ferguson Port,Resnik Beacon"
+ },
+ {
+ "name": "Wakawal",
+ "pos_x": 0.96875,
+ "pos_y": -2.5625,
+ "pos_z": -77.375,
+ "stations": "Olsen Terminal,Virts Gateway,Good Ring,Clark Camp,Quinn Relay"
+ },
+ {
+ "name": "Wakea",
+ "pos_x": 101,
+ "pos_y": -94.34375,
+ "pos_z": 36.15625,
+ "stations": "Alexandra Station,Valdeaguas,Giraud Depot,Goldstein Park"
+ },
+ {
+ "name": "Wakinuke",
+ "pos_x": 136.5625,
+ "pos_y": -22.28125,
+ "pos_z": 65.1875,
+ "stations": "Osterbrock Terminal,Lunan Vision,Lippisch Settlement"
+ },
+ {
+ "name": "Wakinyan",
+ "pos_x": 79,
+ "pos_y": 75.71875,
+ "pos_z": 28.9375,
+ "stations": "Melvill Gateway,Malenchenko Dock,Duke Ring,Daley Holdings,Hoffman Orbital,Garriott Ring,Henize Gateway,Goonan's Folly,Al-Battani Terminal"
+ },
+ {
+ "name": "Wakonoto",
+ "pos_x": 72.78125,
+ "pos_y": 118.25,
+ "pos_z": 19.71875,
+ "stations": "Kanwar Station,Mills City,Deluc Orbital"
+ },
+ {
+ "name": "Wakos",
+ "pos_x": -42.96875,
+ "pos_y": 106.15625,
+ "pos_z": -80.28125,
+ "stations": "Hawkes Station,Birkhoff Settlement,Gulyaev Camp"
+ },
+ {
+ "name": "Wakot",
+ "pos_x": -82.375,
+ "pos_y": 95,
+ "pos_z": 60.5625,
+ "stations": "Rothman Dock,Cady Silo,Biggle Beacon"
+ },
+ {
+ "name": "Wala",
+ "pos_x": 5.9375,
+ "pos_y": -39.03125,
+ "pos_z": -33.53125,
+ "stations": "Titov Dock,Clark Landing"
+ },
+ {
+ "name": "Walakkaits",
+ "pos_x": 134.40625,
+ "pos_y": -128.8125,
+ "pos_z": -73.5,
+ "stations": "Heinemann Stop"
+ },
+ {
+ "name": "Walangama",
+ "pos_x": -65,
+ "pos_y": 50.6875,
+ "pos_z": 96,
+ "stations": "Chwedyk Port,Fourier City"
+ },
+ {
+ "name": "Walbanga",
+ "pos_x": -129.6875,
+ "pos_y": 68.875,
+ "pos_z": 93.21875,
+ "stations": "Barreiros Works,Chaviano Camp"
+ },
+ {
+ "name": "Walgal",
+ "pos_x": 66.3125,
+ "pos_y": -21.03125,
+ "pos_z": 18.09375,
+ "stations": "Carpenter City,Davidson Installation,Low Ring,Bernard Installation,Rescue Ship - Carpenter City"
+ },
+ {
+ "name": "Walgalu",
+ "pos_x": 178.78125,
+ "pos_y": -159.625,
+ "pos_z": 83.59375,
+ "stations": "Kaluta Platform"
+ },
+ {
+ "name": "Walgan",
+ "pos_x": 63.8125,
+ "pos_y": -23.84375,
+ "pos_z": 22.6875,
+ "stations": "McMullen Colony,Garden Refinery"
+ },
+ {
+ "name": "Waljen",
+ "pos_x": 42.59375,
+ "pos_y": -112.46875,
+ "pos_z": 80.46875,
+ "stations": "Emshwiller Survey,Michael Colony"
+ },
+ {
+ "name": "Walka",
+ "pos_x": 26.28125,
+ "pos_y": -72.46875,
+ "pos_z": 63.96875,
+ "stations": "Gregory City,Bainbridge Prospect,Russell Hub,Alvares Orbital,Read Base"
+ },
+ {
+ "name": "Walla",
+ "pos_x": -172.15625,
+ "pos_y": -43.75,
+ "pos_z": -62.6875,
+ "stations": "Eisenstein City,Farrukh Ring,Erdos Refinery"
+ },
+ {
+ "name": "Walla Walla",
+ "pos_x": -31.46875,
+ "pos_y": -95.4375,
+ "pos_z": 62.65625,
+ "stations": "Grant Colony,Kingsbury's Claim"
+ },
+ {
+ "name": "Walladals",
+ "pos_x": -120.625,
+ "pos_y": -22.59375,
+ "pos_z": 125.15625,
+ "stations": "Parry Settlement"
+ },
+ {
+ "name": "Wallangu",
+ "pos_x": 48.875,
+ "pos_y": -90.03125,
+ "pos_z": -16.375,
+ "stations": "Krusvar Settlement,Noli City,Ziemkiewicz Prospect"
+ },
+ {
+ "name": "Wallatu",
+ "pos_x": 77.78125,
+ "pos_y": -188.6875,
+ "pos_z": 108.53125,
+ "stations": "Honda Colony"
+ },
+ {
+ "name": "Wallicheo",
+ "pos_x": 111.4375,
+ "pos_y": -222.71875,
+ "pos_z": -12.40625,
+ "stations": "Hell's Claim"
+ },
+ {
+ "name": "Wallites",
+ "pos_x": 111.6875,
+ "pos_y": -259.125,
+ "pos_z": -22.6875,
+ "stations": "Lane Settlement,Davis Landing,Consolmagno Port"
+ },
+ {
+ "name": "Wallu",
+ "pos_x": -22.46875,
+ "pos_y": -197.90625,
+ "pos_z": -41.1875,
+ "stations": "Carsono Platform,Maughmer Camp"
+ },
+ {
+ "name": "Wallunga",
+ "pos_x": -51.53125,
+ "pos_y": -151.8125,
+ "pos_z": 77.90625,
+ "stations": "Matthews Vision,Cummings Station,Montanari Terminal,Holden Works,Carleson Installation"
+ },
+ {
+ "name": "Wally Bei",
+ "pos_x": -14.875,
+ "pos_y": 7.5,
+ "pos_z": 65.78125,
+ "stations": "Volk Dock,Boas Terminal,Swanson Port,Malerba Orbital"
+ },
+ {
+ "name": "Wally Berod",
+ "pos_x": 162.0625,
+ "pos_y": -173.8125,
+ "pos_z": 127.9375,
+ "stations": "al-Din al-Urdi Prospect"
+ },
+ {
+ "name": "Walmadjari",
+ "pos_x": 134.96875,
+ "pos_y": -95,
+ "pos_z": 125.40625,
+ "stations": "Gulyaev Plant,Draper Platform,Sandage Platform"
+ },
+ {
+ "name": "Walmanglai",
+ "pos_x": -0.15625,
+ "pos_y": 97.59375,
+ "pos_z": -103.9375,
+ "stations": "Lanchester Mine,Culpeper Camp,Fulton Refinery"
+ },
+ {
+ "name": "Walmiki",
+ "pos_x": -1.625,
+ "pos_y": 79.96875,
+ "pos_z": 117.59375,
+ "stations": "Lamarr Plant,Leopold Colony"
+ },
+ {
+ "name": "Walmir",
+ "pos_x": 79.46875,
+ "pos_y": -35.875,
+ "pos_z": -36.21875,
+ "stations": "Evans Orbital"
+ },
+ {
+ "name": "Walmiri",
+ "pos_x": 24.65625,
+ "pos_y": 81.09375,
+ "pos_z": 41.3125,
+ "stations": "Fossum City,Virtanen Hub,Napier Orbital,Yano Observatory,Mitchell Base"
+ },
+ {
+ "name": "Walpi",
+ "pos_x": 38.21875,
+ "pos_y": -26.90625,
+ "pos_z": -47.40625,
+ "stations": "Garan Platform,Thomas Enterprise,Meitner Hangar,Precourt Refinery,Detmer's Folly"
+ },
+ {
+ "name": "Walpiri",
+ "pos_x": 151.34375,
+ "pos_y": -114.71875,
+ "pos_z": 64.84375,
+ "stations": "Lubbock Dock,Hirayama Market"
+ },
+ {
+ "name": "Waluk",
+ "pos_x": -173.0625,
+ "pos_y": 39.46875,
+ "pos_z": -27,
+ "stations": "Darboux Relay,Bradley Laboratory"
+ },
+ {
+ "name": "Walumbhaik",
+ "pos_x": -29.28125,
+ "pos_y": 11.4375,
+ "pos_z": 83.25,
+ "stations": "Hand Dock,Finney Point"
+ },
+ {
+ "name": "Walun",
+ "pos_x": -3.40625,
+ "pos_y": -95.53125,
+ "pos_z": 136.9375,
+ "stations": "Jekhowsky Survey"
+ },
+ {
+ "name": "Walung Yao",
+ "pos_x": 79.09375,
+ "pos_y": -226.0625,
+ "pos_z": -18.59375,
+ "stations": "Valz Point"
+ },
+ {
+ "name": "Wambal",
+ "pos_x": -32.8125,
+ "pos_y": 21.5,
+ "pos_z": 75.0625,
+ "stations": "Culbertson Port,Song Station"
+ },
+ {
+ "name": "Wanagi",
+ "pos_x": 91.21875,
+ "pos_y": -148.4375,
+ "pos_z": 131.46875,
+ "stations": "Espenak Mine"
+ },
+ {
+ "name": "Wanano",
+ "pos_x": 97.53125,
+ "pos_y": -224.6875,
+ "pos_z": 78.375,
+ "stations": "Mayor Mines,Maunder Holdings"
+ },
+ {
+ "name": "Wandarang",
+ "pos_x": -90.625,
+ "pos_y": 113.8125,
+ "pos_z": 56.34375,
+ "stations": "Wilson's Claim,Barreiros Depot,Allen Base"
+ },
+ {
+ "name": "Wandhridja",
+ "pos_x": 69.75,
+ "pos_y": -151.40625,
+ "pos_z": -67.8125,
+ "stations": "Adkins Works,la Cosa's Progress"
+ },
+ {
+ "name": "Wandir",
+ "pos_x": 22.65625,
+ "pos_y": -164.25,
+ "pos_z": 138.5625,
+ "stations": "Kemurdzhian Orbital,Rosenberger Ring,Wegener Hub,Chertovsky's Inheritance,Belyanin's Progress"
+ },
+ {
+ "name": "Wandjera",
+ "pos_x": 14.28125,
+ "pos_y": -12.15625,
+ "pos_z": 153.9375,
+ "stations": "Parsons Station,Tiptree Prospect,Wakata Port,Ivanchenkov Dock,Henslow Refinery"
+ },
+ {
+ "name": "Wandjin",
+ "pos_x": 85.03125,
+ "pos_y": -127.625,
+ "pos_z": 21.96875,
+ "stations": "Vuia Port,Schmidt Terminal,Joseph Delambre Prospect"
+ },
+ {
+ "name": "Wandrama",
+ "pos_x": -90.25,
+ "pos_y": -103.3125,
+ "pos_z": -94.96875,
+ "stations": "MacLean City,Al-Farabi Gateway,Jemison Settlement"
+ },
+ {
+ "name": "Wandu",
+ "pos_x": 164.5,
+ "pos_y": -40.4375,
+ "pos_z": -32.125,
+ "stations": "Dittmar Dock"
+ },
+ {
+ "name": "Wang Dana",
+ "pos_x": 161.71875,
+ "pos_y": 29.5,
+ "pos_z": 72.46875,
+ "stations": "Gibson City,Budarin's Progress,Fleming Orbital,Bernoulli Legacy"
+ },
+ {
+ "name": "Wang Ku",
+ "pos_x": 103.71875,
+ "pos_y": 84.4375,
+ "pos_z": -37.59375,
+ "stations": "Mastracchio Dock,Wang Port,Stanley Point"
+ },
+ {
+ "name": "Wang Walli",
+ "pos_x": 150,
+ "pos_y": 3.5,
+ "pos_z": -24.3125,
+ "stations": "Back Arsenal,Zindell Port,Moon Arena"
+ },
+ {
+ "name": "Wanga",
+ "pos_x": -87.65625,
+ "pos_y": -140.40625,
+ "pos_z": 82.28125,
+ "stations": "Howard Legacy"
+ },
+ {
+ "name": "Wangai",
+ "pos_x": 65.3125,
+ "pos_y": -143.375,
+ "pos_z": 99.03125,
+ "stations": "Harvia Station"
+ },
+ {
+ "name": "Wangakwan",
+ "pos_x": 100.34375,
+ "pos_y": -65.46875,
+ "pos_z": -136.125,
+ "stations": "Onufrienko Port,Archambault Station,Oefelein Station,Jordan Holdings"
+ },
+ {
+ "name": "Wangal",
+ "pos_x": 84.625,
+ "pos_y": -101.40625,
+ "pos_z": -37,
+ "stations": "Rothfuss Orbital,Potagos Port,Resnik Lab,Vries Orbital,Nelder City,Neff Point,Reed Port,Bierce Survey,Garrett Station,Hannu Dock,Henslow Gateway,Stairs Reach,Kozlov Dock,Dedman Station"
+ },
+ {
+ "name": "Wangana",
+ "pos_x": -53.9375,
+ "pos_y": -65.90625,
+ "pos_z": 150.9375,
+ "stations": "Snodgrass Platform,Moon Port,Wnuk-Lipinski's Folly"
+ },
+ {
+ "name": "Wanges",
+ "pos_x": -45.34375,
+ "pos_y": -64.4375,
+ "pos_z": 129.03125,
+ "stations": "Cook's Pride,Ocampo Settlement"
+ },
+ {
+ "name": "Wanggu",
+ "pos_x": -132.71875,
+ "pos_y": 30.96875,
+ "pos_z": -39.59375,
+ "stations": "Qureshi Colony"
+ },
+ {
+ "name": "Wangjel",
+ "pos_x": 12.03125,
+ "pos_y": 154.96875,
+ "pos_z": -67.375,
+ "stations": "Linge Beacon,Brackett Beacon,Staden Holdings"
+ },
+ {
+ "name": "Wangkathaa",
+ "pos_x": -46.46875,
+ "pos_y": 72.25,
+ "pos_z": 85.625,
+ "stations": "Wheelock Plant,Alas Port,Brunner's Inheritance"
+ },
+ {
+ "name": "Wanglang Mu",
+ "pos_x": 126.78125,
+ "pos_y": -192.59375,
+ "pos_z": 115.4375,
+ "stations": "Dunlop Horizons,Fedden Vision,Langley Depot"
+ },
+ {
+ "name": "Wangmin",
+ "pos_x": 123.625,
+ "pos_y": 57.03125,
+ "pos_z": -66.46875,
+ "stations": "Noriega Platform,Diesel Vision"
+ },
+ {
+ "name": "Wangs",
+ "pos_x": 46,
+ "pos_y": 24.1875,
+ "pos_z": -138.375,
+ "stations": "Bolger's Inheritance,Antonio de Andrade's Exile"
+ },
+ {
+ "name": "Wanjan",
+ "pos_x": 66.75,
+ "pos_y": -112.4375,
+ "pos_z": 65.25,
+ "stations": "Weaver Vision,Zwicky Terminal,Oterma's Claim,Nachtigal Survey"
+ },
+ {
+ "name": "Wanjiwalku",
+ "pos_x": -21.59375,
+ "pos_y": 122.71875,
+ "pos_z": 110.03125,
+ "stations": "Malenchenko Terminal,Ford Ring,Ferguson Orbital"
+ },
+ {
+ "name": "Wanjul",
+ "pos_x": 114.28125,
+ "pos_y": -118.875,
+ "pos_z": 127.71875,
+ "stations": "Henderson Station,Anderson Port,Joseph Delambre Terminal,Thorne Settlement,van de Sande Bakhuyzen Bastion"
+ },
+ {
+ "name": "Wanmi",
+ "pos_x": -136.5625,
+ "pos_y": -84.25,
+ "pos_z": -35.8125,
+ "stations": "Bridger Penal colony,Soper Arena"
+ },
+ {
+ "name": "Wannovik",
+ "pos_x": 99.59375,
+ "pos_y": -222.875,
+ "pos_z": 83.375,
+ "stations": "Banno Station,Geller Colony,Whitford Landing,Arkhangelsky Vision"
+ },
+ {
+ "name": "Wapiety",
+ "pos_x": 84.1875,
+ "pos_y": -7.4375,
+ "pos_z": 165.71875,
+ "stations": "Crumey Refinery,Barton Enterprise"
+ },
+ {
+ "name": "Wapis",
+ "pos_x": 105.59375,
+ "pos_y": -105.21875,
+ "pos_z": 116.78125,
+ "stations": "Lagadha Terminal,Edwards Dock,Wetherill Vision,Al Sufi Penal colony"
+ },
+ {
+ "name": "Wapitis",
+ "pos_x": 120,
+ "pos_y": 23.90625,
+ "pos_z": 44.53125,
+ "stations": "Andree Port,Brust Silo,Zetford Terminal"
+ },
+ {
+ "name": "Wapiya",
+ "pos_x": 54.21875,
+ "pos_y": -154.84375,
+ "pos_z": 30.625,
+ "stations": "Brothers Vision,Reber Orbital,Brera Beacon,Lefschetz Base,Lemaitre Ring"
+ },
+ {
+ "name": "Waq",
+ "pos_x": 86.90625,
+ "pos_y": -130.28125,
+ "pos_z": 98.84375,
+ "stations": "Fischer Orbital,Mandel Terminal,Robert Station,Utley's Inheritance,Bainbridge Station,Lanier Survey"
+ },
+ {
+ "name": "Waragwaa",
+ "pos_x": 102.5625,
+ "pos_y": -179.59375,
+ "pos_z": 115.5,
+ "stations": "Powers Retreat"
+ },
+ {
+ "name": "Warahati",
+ "pos_x": 85.3125,
+ "pos_y": 94.21875,
+ "pos_z": -38.875,
+ "stations": "Linenger Horizons,Bhabha Vision"
+ },
+ {
+ "name": "Warakmbut",
+ "pos_x": 115.03125,
+ "pos_y": -240.4375,
+ "pos_z": 38.46875,
+ "stations": "Brahmagupta Retreat"
+ },
+ {
+ "name": "Waramunava",
+ "pos_x": -11.34375,
+ "pos_y": -74.40625,
+ "pos_z": 112.65625,
+ "stations": "Shkaplerov Port"
+ },
+ {
+ "name": "Warao",
+ "pos_x": 4.15625,
+ "pos_y": 95.03125,
+ "pos_z": 95.15625,
+ "stations": "Bamford Orbital,Sullivan Terminal,Roosa Hub,Avogadro Orbital,Vishweswarayya Terminal,Dobzhansky Terminal,Payton Enterprise,Stevens' Progress,Barcelo Settlement,Macdonald's Progress,Godwin's Inheritance"
+ },
+ {
+ "name": "Waras",
+ "pos_x": 161.15625,
+ "pos_y": -121.46875,
+ "pos_z": -59.53125,
+ "stations": "Herreshoff Penal colony,Rizvi Terminal,Smoot Oasis"
+ },
+ {
+ "name": "Waraswarre",
+ "pos_x": 35.90625,
+ "pos_y": 17.40625,
+ "pos_z": 165.875,
+ "stations": "Mendeleev Enterprise,Hale Enterprise,Afanasyev Station,Lopez de Haro Beacon,Lyakhov Enterprise"
+ },
+ {
+ "name": "Warayu",
+ "pos_x": 98.25,
+ "pos_y": 124.8125,
+ "pos_z": 6.375,
+ "stations": "Clervoy Port"
+ },
+ {
+ "name": "Wardal",
+ "pos_x": -82.9375,
+ "pos_y": 40.6875,
+ "pos_z": 29.4375,
+ "stations": "Malyshev Installation,Goeschke Colony,Cugnot's Claim"
+ },
+ {
+ "name": "Wardaliti",
+ "pos_x": 71.0625,
+ "pos_y": -16.71875,
+ "pos_z": -15,
+ "stations": "Szameit Enterprise,Pribylov Oasis,Hunt City,Napier Hub,Morgan Station,Griffith Terminal,Scalzi Depot,Capek Enterprise,Dozois Gateway,Vasilyev Survey,Gerrold Ring,Hovell Escape,Roddenberry Vision"
+ },
+ {
+ "name": "Wardh",
+ "pos_x": 205.375,
+ "pos_y": -173.1875,
+ "pos_z": 28.75,
+ "stations": "Battani Depot,Herreshoff Depot"
+ },
+ {
+ "name": "Wardhara",
+ "pos_x": 82.1875,
+ "pos_y": -137.15625,
+ "pos_z": 56.21875,
+ "stations": "Miller Base,Ludwig Struve Prospect,Stechkin Colony"
+ },
+ {
+ "name": "Wardhr",
+ "pos_x": 23.96875,
+ "pos_y": -184.875,
+ "pos_z": 56.125,
+ "stations": "Moffat Ring,van den Bergh Vision,Herschel City,Yize Station"
+ },
+ {
+ "name": "Wardins",
+ "pos_x": 33.375,
+ "pos_y": -115.34375,
+ "pos_z": -84,
+ "stations": "van de Hulst Vision,Whittle Relay,Patry Hub"
+ },
+ {
+ "name": "Wardok",
+ "pos_x": 81.8125,
+ "pos_y": -119.28125,
+ "pos_z": -29.8125,
+ "stations": "Wegener Point"
+ },
+ {
+ "name": "Wardovik",
+ "pos_x": -55.75,
+ "pos_y": -144.40625,
+ "pos_z": 1.40625,
+ "stations": "Piccard Outpost"
+ },
+ {
+ "name": "Wargis",
+ "pos_x": 16.8125,
+ "pos_y": -95.25,
+ "pos_z": -73.9375,
+ "stations": "Gehry Port,Mooz Terminal,Karlsefni Plant,Phillips Dock,Gold Prospect"
+ },
+ {
+ "name": "Wari",
+ "pos_x": -135.5,
+ "pos_y": 52.15625,
+ "pos_z": 8.6875,
+ "stations": "Schrodinger Dock,Haber Ring,Dorsey Landing,Walheim Ring,Gagarin Orbital,Mohmand Orbital,Kube-McDowell Prospect,Tepper Beacon,Sarafanov Settlement"
+ },
+ {
+ "name": "Wariangga",
+ "pos_x": -118.90625,
+ "pos_y": 118.46875,
+ "pos_z": 24.21875,
+ "stations": "Rose Mine,Guerrero Base"
+ },
+ {
+ "name": "Warians",
+ "pos_x": 155.40625,
+ "pos_y": -18.09375,
+ "pos_z": 61.78125,
+ "stations": "Caidin Legacy,McMahon Lab"
+ },
+ {
+ "name": "Waribe",
+ "pos_x": -80.75,
+ "pos_y": 33.75,
+ "pos_z": 73.0625,
+ "stations": "Kubasov Settlement,Curbeam Vision,Schmitt Camp"
+ },
+ {
+ "name": "Waribroci",
+ "pos_x": 69.0625,
+ "pos_y": -142.53125,
+ "pos_z": 135.15625,
+ "stations": "Gurevich Colony"
+ },
+ {
+ "name": "Waricare",
+ "pos_x": -57.4375,
+ "pos_y": 44.5625,
+ "pos_z": 55.5625,
+ "stations": "Sturckow Vision,Hutton Colony,Popov Bastion,Brongniart Base"
+ },
+ {
+ "name": "Warici",
+ "pos_x": -13.40625,
+ "pos_y": -68.21875,
+ "pos_z": -136.09375,
+ "stations": "Roberts Penal colony,Nye Station,Werner von Siemens Point"
+ },
+ {
+ "name": "Warigua",
+ "pos_x": -14.625,
+ "pos_y": -36.25,
+ "pos_z": 124.90625,
+ "stations": "Alexandria City,Weyn Co-operative,Popper Enterprise,Pike Horizons"
+ },
+ {
+ "name": "Warii",
+ "pos_x": 86,
+ "pos_y": -234.59375,
+ "pos_z": 29.34375,
+ "stations": "Pond Port,Roemer Port"
+ },
+ {
+ "name": "Warimpe",
+ "pos_x": 125.09375,
+ "pos_y": -130.875,
+ "pos_z": -86.125,
+ "stations": "Baliunas Platform"
+ },
+ {
+ "name": "Warinegana",
+ "pos_x": -1.8125,
+ "pos_y": -21.53125,
+ "pos_z": 114.625,
+ "stations": "Morrison Colony"
+ },
+ {
+ "name": "Waringpo",
+ "pos_x": 7.4375,
+ "pos_y": 120.5625,
+ "pos_z": -115.40625,
+ "stations": "Tolstoi Camp,Silves Point,Liouville's Progress"
+ },
+ {
+ "name": "Warkan",
+ "pos_x": -32.625,
+ "pos_y": -80,
+ "pos_z": 56.75,
+ "stations": "Malenchenko Ring,Crown Keep"
+ },
+ {
+ "name": "Warkawa",
+ "pos_x": -103.53125,
+ "pos_y": -46.3125,
+ "pos_z": 100.1875,
+ "stations": "Scott Settlement,Kidman Enterprise,Ray Hub"
+ },
+ {
+ "name": "Warkin",
+ "pos_x": -72.75,
+ "pos_y": 29.21875,
+ "pos_z": 86.84375,
+ "stations": "Garratt Settlement,Delbruck Oasis"
+ },
+ {
+ "name": "Warkushanui",
+ "pos_x": -42.625,
+ "pos_y": -85.125,
+ "pos_z": 6.25,
+ "stations": "Coats Hub,Gurragchaa Gateway,Serre Refinery,Marconi Station,Anning Port,Brunner's Progress,Fraser's Inheritance,Cassidy Hub,Beregovoi Orbital"
+ },
+ {
+ "name": "Warkushodi",
+ "pos_x": -119.625,
+ "pos_y": 16.46875,
+ "pos_z": 23.84375,
+ "stations": "Bhabha Camp,Volkov Colony"
+ },
+ {
+ "name": "Warla",
+ "pos_x": -121.0625,
+ "pos_y": -103.5,
+ "pos_z": 37.84375,
+ "stations": "Robinson Landing,Dayuan Landing"
+ },
+ {
+ "name": "Warlaworka",
+ "pos_x": 12.125,
+ "pos_y": -124.25,
+ "pos_z": -24.78125,
+ "stations": "Hughes Mines"
+ },
+ {
+ "name": "Warnates",
+ "pos_x": -46.1875,
+ "pos_y": -4.375,
+ "pos_z": -67.65625,
+ "stations": "Bursch Terminal,Skripochka Port,Yurchikhin Terminal,Herschel Orbital,Blackwell Terminal,Guest Enterprise,Berezin's Inheritance,Laing Terminal,Kuipers Orbital,Readdy Orbital,Ross Orbital"
+ },
+ {
+ "name": "Warninohan",
+ "pos_x": 20.125,
+ "pos_y": -26.25,
+ "pos_z": 73.5,
+ "stations": "Fraas Gateway,Berezovoy Terminal,Bosch City,Haxel Ring,Atkov Prospect,Gromov Refinery,Babbage City,Rominger Gateway"
+ },
+ {
+ "name": "Warnones",
+ "pos_x": -92.40625,
+ "pos_y": 32.0625,
+ "pos_z": -86.1875,
+ "stations": "Humboldt Station,Lousma Port,Brunel Colony,Evans Palace,Meitner Installation,Bugrov Refinery"
+ },
+ {
+ "name": "Warnsaxa",
+ "pos_x": -137.59375,
+ "pos_y": 6.28125,
+ "pos_z": 64.53125,
+ "stations": "Wyndham Station,Barth Point,Dorsey's Progress"
+ },
+ {
+ "name": "Warra",
+ "pos_x": -2.34375,
+ "pos_y": 72.25,
+ "pos_z": 114.1875,
+ "stations": "Gidzenko Terminal"
+ },
+ {
+ "name": "Warrahgnook",
+ "pos_x": -5.875,
+ "pos_y": 29.375,
+ "pos_z": 143.34375,
+ "stations": "Carlisle Port,Laing Works"
+ },
+ {
+ "name": "Warrapatis",
+ "pos_x": 148,
+ "pos_y": -53.375,
+ "pos_z": 92.65625,
+ "stations": "Stephan Vision,Harding Base"
+ },
+ {
+ "name": "Warri",
+ "pos_x": 130.5625,
+ "pos_y": 25.71875,
+ "pos_z": -76.90625,
+ "stations": "Stross Beacon,Stjepan Seljan Relay,Weinbaum Relay"
+ },
+ {
+ "name": "Waru",
+ "pos_x": -4.71875,
+ "pos_y": 59.78125,
+ "pos_z": 37.25,
+ "stations": "Sylvester Installation,Neville's Inheritance,Skolem Colony,Hawke Penal colony"
+ },
+ {
+ "name": "Warundecab",
+ "pos_x": -53.625,
+ "pos_y": -166.21875,
+ "pos_z": -13.46875,
+ "stations": "Caselberg Vision,Narita Dock"
+ },
+ {
+ "name": "Warungkambo",
+ "pos_x": -104.59375,
+ "pos_y": 60.40625,
+ "pos_z": 60,
+ "stations": "Lem Horizons"
+ },
+ {
+ "name": "Waruta",
+ "pos_x": 85.46875,
+ "pos_y": 14.21875,
+ "pos_z": 115.6875,
+ "stations": "Darwin Point,Mallory Oasis"
+ },
+ {
+ "name": "Waruts",
+ "pos_x": 42.9375,
+ "pos_y": 9.34375,
+ "pos_z": -83.75,
+ "stations": "Jenner Hub,Henricks Orbital,Burbank Ring,Hertz Enterprise,Great Landing,Seega Orbital,Feoktistov Palace"
+ },
+ {
+ "name": "Warwa",
+ "pos_x": 100.6875,
+ "pos_y": -64.625,
+ "pos_z": 96.21875,
+ "stations": "Stevenson Hub,Levinson Landing,Fukushima Dock,Otus Dock"
+ },
+ {
+ "name": "Warwad",
+ "pos_x": 64.1875,
+ "pos_y": 130.40625,
+ "pos_z": -126.6875,
+ "stations": "Johnson Reformatory,Yourkevitch Prospect"
+ },
+ {
+ "name": "Warwal",
+ "pos_x": -84.125,
+ "pos_y": 0.65625,
+ "pos_z": 50.28125,
+ "stations": "Buchli City,Cogswell Hub,Haxel Terminal,Bierce Hub,Cole Holdings,Wheelock Hub,Grechko Dock,McMonagle Orbital,Ellern Palace"
+ },
+ {
+ "name": "Warwarindji",
+ "pos_x": 23.625,
+ "pos_y": 52.34375,
+ "pos_z": -170.6875,
+ "stations": "Allen Enterprise,Barton Enterprise,Gaspar de Portola Hub"
+ },
+ {
+ "name": "Wasat",
+ "pos_x": 16.3125,
+ "pos_y": 17.125,
+ "pos_z": -55.65625,
+ "stations": "White Terminal,Elwood Camp,Behring Point,Bombelli Dock,Ferguson Prospect,Cenker Arena"
+ },
+ {
+ "name": "Wasenateno",
+ "pos_x": 157.625,
+ "pos_y": -111.0625,
+ "pos_z": 46.875,
+ "stations": "Furukawa Mines,Beshore Dock"
+ },
+ {
+ "name": "Wasenensei",
+ "pos_x": 88.625,
+ "pos_y": -14.8125,
+ "pos_z": 71.4375,
+ "stations": "Anderson Lab,Pilyugin Depot"
+ },
+ {
+ "name": "Wasens",
+ "pos_x": 159.46875,
+ "pos_y": -179.6875,
+ "pos_z": 57.8125,
+ "stations": "Hardy Terminal"
+ },
+ {
+ "name": "Wasetae",
+ "pos_x": -37.125,
+ "pos_y": -67.9375,
+ "pos_z": 47,
+ "stations": "Babcock Mines,Borman Colony,Bernoulli Laboratory"
+ },
+ {
+ "name": "Washa",
+ "pos_x": 42.4375,
+ "pos_y": -8.875,
+ "pos_z": 156.84375,
+ "stations": "Bertin Platform,Ricci Penal colony"
+ },
+ {
+ "name": "Washi",
+ "pos_x": 15.1875,
+ "pos_y": -252.5,
+ "pos_z": 42.25,
+ "stations": "Shiner Depot,Tusi Oasis,Uto Landing"
+ },
+ {
+ "name": "Washika",
+ "pos_x": 15.8125,
+ "pos_y": -55.0625,
+ "pos_z": 120.09375,
+ "stations": "Mieville Hub"
+ },
+ {
+ "name": "Washiri",
+ "pos_x": 36.09375,
+ "pos_y": -199.0625,
+ "pos_z": 80.71875,
+ "stations": "Brillant's Claim,Zenbei Outpost"
+ },
+ {
+ "name": "Washodia",
+ "pos_x": -144.34375,
+ "pos_y": 45.53125,
+ "pos_z": 48.53125,
+ "stations": "Rayhan al-Biruni Port,Norton Ring,Ordway City,Shaver Beacon,Carter Enterprise"
+ },
+ {
+ "name": "Wat Saman",
+ "pos_x": -140.9375,
+ "pos_y": -4.84375,
+ "pos_z": -22.96875,
+ "stations": "Hill Prospect,Perrin Plant,Zhigang Colony"
+ },
+ {
+ "name": "Wat Yu",
+ "pos_x": -27.09375,
+ "pos_y": 6.15625,
+ "pos_z": 36.4375,
+ "stations": "King Oasis,Musgrave Station,Cameron Gateway,Hinz Camp"
+ },
+ {
+ "name": "Watha",
+ "pos_x": -24.5,
+ "pos_y": 34,
+ "pos_z": 113.625,
+ "stations": "Szilard Depot,Binnie Market,Warner Holdings,Stapledon Landing,Adams Holdings"
+ },
+ {
+ "name": "Wathi",
+ "pos_x": -68,
+ "pos_y": -54.71875,
+ "pos_z": 52.1875,
+ "stations": "Chomsky Horizons,Neumann Station"
+ },
+ {
+ "name": "Wathiparian",
+ "pos_x": -22.46875,
+ "pos_y": 13.78125,
+ "pos_z": -93.34375,
+ "stations": "Kummer Terminal,Przhevalsky Port,Reightler Relay,Kelleam City,Knight Station,McCarthy Holdings,Mitchell Orbital,Raleigh Horizons,Sabine Hub,Love Base"
+ },
+ {
+ "name": "Wathlanukh",
+ "pos_x": -149.21875,
+ "pos_y": 5.25,
+ "pos_z": -60.6875,
+ "stations": "Banks Port"
+ },
+ {
+ "name": "Watilo",
+ "pos_x": 66.0625,
+ "pos_y": 91.3125,
+ "pos_z": 2.6875,
+ "stations": "Crown Base,Gooch Landing,Darwin Settlement"
+ },
+ {
+ "name": "Watis",
+ "pos_x": -80.34375,
+ "pos_y": 0.09375,
+ "pos_z": -104.03125,
+ "stations": "Thomas Settlement"
+ },
+ {
+ "name": "Watistha",
+ "pos_x": -120.96875,
+ "pos_y": 100.5625,
+ "pos_z": -60.28125,
+ "stations": "Ellison Beacon,Bentham Mines"
+ },
+ {
+ "name": "Watiwati",
+ "pos_x": -52.875,
+ "pos_y": 51.875,
+ "pos_z": -98.0625,
+ "stations": "Klein Terminal,Cantor Installation,Sabine Survey"
+ },
+ {
+ "name": "Wattragwa",
+ "pos_x": -2.375,
+ "pos_y": -139.28125,
+ "pos_z": 30.25,
+ "stations": "Tully Port"
+ },
+ {
+ "name": "Wattunukam",
+ "pos_x": -119.875,
+ "pos_y": -90.21875,
+ "pos_z": 9.96875,
+ "stations": "Drew Base,Redi Mine,Kekule Camp"
+ },
+ {
+ "name": "Wattva",
+ "pos_x": -72.4375,
+ "pos_y": 45.90625,
+ "pos_z": 88.0625,
+ "stations": "Clervoy Settlement,Chu Outpost"
+ },
+ {
+ "name": "Wattvasika",
+ "pos_x": -18.5625,
+ "pos_y": 60.28125,
+ "pos_z": 87.34375,
+ "stations": "Duke Terminal,Anderson Station,Comino Orbital"
+ },
+ {
+ "name": "Waukan",
+ "pos_x": -71.40625,
+ "pos_y": 113.84375,
+ "pos_z": 50.125,
+ "stations": "Foden Base,Griffith Installation"
+ },
+ {
+ "name": "Wauksarra",
+ "pos_x": -90.28125,
+ "pos_y": -110.28125,
+ "pos_z": 4.59375,
+ "stations": "Stewart Relay"
+ },
+ {
+ "name": "Waventeno",
+ "pos_x": 24.375,
+ "pos_y": -174.03125,
+ "pos_z": -51.21875,
+ "stations": "Anastase Perrotin Mines"
+ },
+ {
+ "name": "Wawal",
+ "pos_x": 35.5625,
+ "pos_y": -1.3125,
+ "pos_z": -93.25,
+ "stations": "Spedding Enterprise,Altman Port"
+ },
+ {
+ "name": "Wayahukulha",
+ "pos_x": 95.28125,
+ "pos_y": -195.8125,
+ "pos_z": 18.125,
+ "stations": "Herzog Vision,Walotsky Prospect"
+ },
+ {
+ "name": "Wayaliti",
+ "pos_x": -3.84375,
+ "pos_y": -33.6875,
+ "pos_z": -83.40625,
+ "stations": "Plexico Vision"
+ },
+ {
+ "name": "Wayan",
+ "pos_x": 98.90625,
+ "pos_y": -201.75,
+ "pos_z": -55.78125,
+ "stations": "Hawker Depot,West Laboratory"
+ },
+ {
+ "name": "Wayano",
+ "pos_x": -94.03125,
+ "pos_y": -81.28125,
+ "pos_z": -59.46875,
+ "stations": "Neville Station,Darboux Town,Carlisle Port,Steiner Settlement,Krylov Palace"
+ },
+ {
+ "name": "Wayica",
+ "pos_x": 19.96875,
+ "pos_y": -210.6875,
+ "pos_z": -47.09375,
+ "stations": "Osterbrock Landing"
+ },
+ {
+ "name": "Wayilwa",
+ "pos_x": -48.46875,
+ "pos_y": 44.1875,
+ "pos_z": -136.125,
+ "stations": "Shonin Gateway,Oliver Vision"
+ },
+ {
+ "name": "Wayimstii",
+ "pos_x": -16.96875,
+ "pos_y": 16.78125,
+ "pos_z": 134.75,
+ "stations": "Macquorn Rankine Camp"
+ },
+ {
+ "name": "Wayutabal",
+ "pos_x": 101.3125,
+ "pos_y": -81.90625,
+ "pos_z": -9.09375,
+ "stations": "Babakin Port,Kaiser Terminal,Reber Station,Weaver Ring,Truly Holdings,Semeonis Refinery,Valigursky Base"
+ },
+ {
+ "name": "Wazn",
+ "pos_x": 68.375,
+ "pos_y": -39.21875,
+ "pos_z": -37.25,
+ "stations": "Bulleid Survey,Jernigan Horizons,Auer Horizons"
+ },
+ {
+ "name": "We Gu",
+ "pos_x": 80.21875,
+ "pos_y": 78.0625,
+ "pos_z": -1.40625,
+ "stations": "Marley Plant"
+ },
+ {
+ "name": "We Guan",
+ "pos_x": 49.625,
+ "pos_y": -29.8125,
+ "pos_z": -99.90625,
+ "stations": "Schottky Port,Yaping Survey"
+ },
+ {
+ "name": "We Jenu",
+ "pos_x": 149.96875,
+ "pos_y": 23.1875,
+ "pos_z": 24.5625,
+ "stations": "Caryanda Port,Steinmuller Beacon,Nagata Plant"
+ },
+ {
+ "name": "We Jenuteki",
+ "pos_x": 63.90625,
+ "pos_y": 120.15625,
+ "pos_z": 72.875,
+ "stations": "Dampier Installation,Birkhoff Colony"
+ },
+ {
+ "name": "Wei Chang",
+ "pos_x": 120.8125,
+ "pos_y": -44.28125,
+ "pos_z": 58.65625,
+ "stations": "Ray City,Barnes Horizons,Corte-Real Base,Bohr Settlement,Wyndham Horizons"
+ },
+ {
+ "name": "Wei Hsia",
+ "pos_x": 60.3125,
+ "pos_y": -193.21875,
+ "pos_z": 104.5625,
+ "stations": "Dumont Hub,Leinster Point"
+ },
+ {
+ "name": "Wei Hua",
+ "pos_x": -59.625,
+ "pos_y": 20.78125,
+ "pos_z": -77.6875,
+ "stations": "Darwin Hub,Wang Prospect,Kirchoff Barracks"
+ },
+ {
+ "name": "Wei Jung",
+ "pos_x": -124.71875,
+ "pos_y": 14.28125,
+ "pos_z": -29.1875,
+ "stations": "Wandrei Enterprise,Bloch Gateway,Mitchison City,Shumil Horizons,Alvares Depot"
+ },
+ {
+ "name": "Wei Tie Yu",
+ "pos_x": 137.125,
+ "pos_y": 59.40625,
+ "pos_z": 76,
+ "stations": "Caselberg's Exile"
+ },
+ {
+ "name": "Weleserme",
+ "pos_x": 90.75,
+ "pos_y": 7.8125,
+ "pos_z": 55.5,
+ "stations": "Harrison Settlement"
+ },
+ {
+ "name": "Weleutaheim",
+ "pos_x": -8.4375,
+ "pos_y": 95.21875,
+ "pos_z": 134.5,
+ "stations": "Chwedyk Colony"
+ },
+ {
+ "name": "Wembal",
+ "pos_x": -119.09375,
+ "pos_y": -67.6875,
+ "pos_z": -14.375,
+ "stations": "Pullman Settlement"
+ },
+ {
+ "name": "Wen",
+ "pos_x": 139.125,
+ "pos_y": -202.90625,
+ "pos_z": -60.40625,
+ "stations": "Faye Ring,Savorgnan de Brazza's Inheritance,Aller Hub,Anderson Station,McDevitt Bastion"
+ },
+ {
+ "name": "Wen Bosnja",
+ "pos_x": 58.40625,
+ "pos_y": 109,
+ "pos_z": -14.75,
+ "stations": "Martins Ring,Nagata Mines,Sinisalo Vision,Strekalov Laboratory"
+ },
+ {
+ "name": "Wen Chang",
+ "pos_x": -52.78125,
+ "pos_y": -210.53125,
+ "pos_z": 63.40625,
+ "stations": "Trumpler Mines,Hunt Silo,Zenbei Legacy"
+ },
+ {
+ "name": "Wen Chayuse",
+ "pos_x": 0.25,
+ "pos_y": -59.78125,
+ "pos_z": 78.09375,
+ "stations": "Naburimannu Terminal"
+ },
+ {
+ "name": "Wen Cher",
+ "pos_x": -22.71875,
+ "pos_y": -44.75,
+ "pos_z": 15.40625,
+ "stations": "O'Connor Installation,Sellers Works"
+ },
+ {
+ "name": "Wen Kuanei",
+ "pos_x": -20.25,
+ "pos_y": 104.40625,
+ "pos_z": 95.90625,
+ "stations": "Niven Relay,Hutchinson Landing,Smith Installation,Nikitin Refinery"
+ },
+ {
+ "name": "Wen Salla",
+ "pos_x": -102.03125,
+ "pos_y": -143.15625,
+ "pos_z": 77.96875,
+ "stations": "McDonald Platform"
+ },
+ {
+ "name": "Wen Tsao Gu",
+ "pos_x": -48.875,
+ "pos_y": 104.875,
+ "pos_z": 19.03125,
+ "stations": "Oxley Dock,Dayuan Terminal,Ivanov Holdings,Kroehl Dock"
+ },
+ {
+ "name": "Wena",
+ "pos_x": -79.0625,
+ "pos_y": 118.46875,
+ "pos_z": 16.875,
+ "stations": "Huberath Dock,Resnick Dock"
+ },
+ {
+ "name": "Wenadou",
+ "pos_x": 9.375,
+ "pos_y": -140.34375,
+ "pos_z": -61.8125,
+ "stations": "Wetherill's Inheritance"
+ },
+ {
+ "name": "Wenda",
+ "pos_x": -68.65625,
+ "pos_y": 73.53125,
+ "pos_z": -73.4375,
+ "stations": "Alexeyev Port,Rucker Orbital,Converse Port,McDevitt Landing,Smith Terminal,Abnett Station,Sacco Depot"
+ },
+ {
+ "name": "Wendeca Ja",
+ "pos_x": 89.4375,
+ "pos_y": 145.75,
+ "pos_z": 24.34375,
+ "stations": "Searfoss Enterprise,Cook's Progress"
+ },
+ {
+ "name": "Wendigo",
+ "pos_x": -91.09375,
+ "pos_y": -138.8125,
+ "pos_z": 56.34375,
+ "stations": "Hausdorff Beacon"
+ },
+ {
+ "name": "Wenha",
+ "pos_x": 103.1875,
+ "pos_y": 103.5,
+ "pos_z": 40.71875,
+ "stations": "Brahe Terminal,Rashid Landing"
+ },
+ {
+ "name": "Wenhilo",
+ "pos_x": 41.78125,
+ "pos_y": -66.9375,
+ "pos_z": 125.0625,
+ "stations": "van Houten Station,Howe Holdings,Escobar's Folly,Killough Installation"
+ },
+ {
+ "name": "Wenufo",
+ "pos_x": 135.90625,
+ "pos_y": -115.25,
+ "pos_z": 121.59375,
+ "stations": "Kawanishi Landing,Wasden Dock"
+ },
+ {
+ "name": "Wepaw",
+ "pos_x": 95.125,
+ "pos_y": -2.90625,
+ "pos_z": 27.34375,
+ "stations": "Akers Terminal"
+ },
+ {
+ "name": "Wepawegana",
+ "pos_x": 77.75,
+ "pos_y": -100.5625,
+ "pos_z": 40.59375,
+ "stations": "Pogson Terminal,Wendelin Vision,Kuchner Vision,Dunlop Bastion"
+ },
+ {
+ "name": "Weramananda",
+ "pos_x": 170.03125,
+ "pos_y": -95.46875,
+ "pos_z": 111,
+ "stations": "Petlyakov Vision,Park Depot"
+ },
+ {
+ "name": "Werapana",
+ "pos_x": -28.09375,
+ "pos_y": -24.5625,
+ "pos_z": -134.625,
+ "stations": "Bethke Ring,Baydukov Orbital,White Vision,Baudin Enterprise,Lethem Enterprise,Lerman Port,George Depot"
+ },
+ {
+ "name": "Weredawasi",
+ "pos_x": -129.90625,
+ "pos_y": -46.5,
+ "pos_z": -104,
+ "stations": "Vance Hub,Alvarez de Pineda Terminal"
+ },
+ {
+ "name": "Wereti",
+ "pos_x": -51.09375,
+ "pos_y": -182.25,
+ "pos_z": -28.59375,
+ "stations": "Romer Survey"
+ },
+ {
+ "name": "Weti",
+ "pos_x": 50.46875,
+ "pos_y": -212.53125,
+ "pos_z": -7.0625,
+ "stations": "Chernykh Station,Plaskett Enterprise,Moon Prospect"
+ },
+ {
+ "name": "Weywisha",
+ "pos_x": -2.53125,
+ "pos_y": 138,
+ "pos_z": 19.65625,
+ "stations": "Berliner Gateway,Willis Base"
+ },
+ {
+ "name": "Weywoth",
+ "pos_x": 99.4375,
+ "pos_y": 13.3125,
+ "pos_z": -28.03125,
+ "stations": "Brillant Dock,Wollheim Prospect"
+ },
+ {
+ "name": "Weywotya",
+ "pos_x": 38.125,
+ "pos_y": -57.0625,
+ "pos_z": -61.40625,
+ "stations": "Aubakirov Colony,Babbage Vision"
+ },
+ {
+ "name": "Whadicates",
+ "pos_x": 98.9375,
+ "pos_y": -95.59375,
+ "pos_z": 81.8125,
+ "stations": "Payne-Gaposchkin Camp"
+ },
+ {
+ "name": "Whadjuk",
+ "pos_x": -98.5625,
+ "pos_y": 28.125,
+ "pos_z": 127.1875,
+ "stations": "Potrykus Mine,Sharipov Outpost,Davy's Progress"
+ },
+ {
+ "name": "Whadros",
+ "pos_x": -55.15625,
+ "pos_y": 94.15625,
+ "pos_z": 169.6875,
+ "stations": "Lie's Inheritance,Lundwall Dock"
+ },
+ {
+ "name": "Whadru",
+ "pos_x": -104.4375,
+ "pos_y": 93.5625,
+ "pos_z": 60.125,
+ "stations": "Archambault Horizons,Banks Landing"
+ },
+ {
+ "name": "Wheelg",
+ "pos_x": 131.65625,
+ "pos_y": -154.25,
+ "pos_z": -42.625,
+ "stations": "Greene Dock"
+ },
+ {
+ "name": "Wheemehimo",
+ "pos_x": 31.75,
+ "pos_y": -79.6875,
+ "pos_z": 146.28125,
+ "stations": "Vaisala Penal colony,van den Bergh Point"
+ },
+ {
+ "name": "Wheemete",
+ "pos_x": 145.125,
+ "pos_y": -140.0625,
+ "pos_z": 131.96875,
+ "stations": "Eisinga Enterprise,Stanley Vision,Mustelin Penal colony,Shirazi Dock"
+ },
+ {
+ "name": "Wheemez",
+ "pos_x": 78.4375,
+ "pos_y": -144.71875,
+ "pos_z": 60.375,
+ "stations": "Bradfield Station,Shklovsky Station"
+ },
+ {
+ "name": "White Sun",
+ "pos_x": -9521,
+ "pos_y": -916.125,
+ "pos_z": 19853.1875,
+ "stations": "Eavesdown Docks"
+ },
+ {
+ "name": "Wi",
+ "pos_x": 13.53125,
+ "pos_y": 25.6875,
+ "pos_z": -48.15625,
+ "stations": "Salgari Port,Brothers Hub,Wilson Station,Wells Enterprise"
+ },
+ {
+ "name": "Wich",
+ "pos_x": -115.21875,
+ "pos_y": 58.75,
+ "pos_z": 79.78125,
+ "stations": "Manning Enterprise,Norton Park,Lambert City,Paulo da Gama Penal colony,Haber Lab"
+ },
+ {
+ "name": "Wichaimi",
+ "pos_x": 15.5,
+ "pos_y": -141.75,
+ "pos_z": -21.1875,
+ "stations": "Brunner Ring,Ziljak Base,Smirnova Orbital,al-Din al-Urdi Port,Allen St. John Port,Hardy Observatory,Berkey Base,Houtman Silo,Takamizawa Hub,Pickering Port"
+ },
+ {
+ "name": "Wichsena",
+ "pos_x": 91.625,
+ "pos_y": -134.625,
+ "pos_z": 154.3125,
+ "stations": "Hughes Mines,Bothezat Enterprise,Alten Installation"
+ },
+ {
+ "name": "Widjabihiko",
+ "pos_x": -15.25,
+ "pos_y": -171.21875,
+ "pos_z": -24.625,
+ "stations": "Watanabe Dock,Thompson Vision,McKean Installation,Hale Vision"
+ },
+ {
+ "name": "Widjalams",
+ "pos_x": 6,
+ "pos_y": -93.3125,
+ "pos_z": 129.28125,
+ "stations": "Gold Prospect,Lupoff Plant,McNaught Dock"
+ },
+ {
+ "name": "Widjalp",
+ "pos_x": -131.1875,
+ "pos_y": -0.53125,
+ "pos_z": 78.84375,
+ "stations": "Koontz's Claim"
+ },
+ {
+ "name": "Widjangkuma",
+ "pos_x": -115.09375,
+ "pos_y": 19,
+ "pos_z": 36.96875,
+ "stations": "Kent Survey,Janifer Dock"
+ },
+ {
+ "name": "Widow's Light",
+ "pos_x": 33.78125,
+ "pos_y": 41.4375,
+ "pos_z": 72,
+ "stations": "Pasteur Orbital,Fraley Gateway,Sauma Settlement,Carter Hub"
+ },
+ {
+ "name": "Wika Mu",
+ "pos_x": 124.28125,
+ "pos_y": 20.40625,
+ "pos_z": -59.5625,
+ "stations": "Onufrienko's Inheritance,Harbaugh Dock,Borman Port"
+ },
+ {
+ "name": "Wikalar",
+ "pos_x": 164.21875,
+ "pos_y": -125.46875,
+ "pos_z": -54.375,
+ "stations": "Winthrop Mine"
+ },
+ {
+ "name": "Wikanawal",
+ "pos_x": -99.375,
+ "pos_y": -36.9375,
+ "pos_z": 62.71875,
+ "stations": "Schwann Hub,O'Brien Keep"
+ },
+ {
+ "name": "Wikatabar",
+ "pos_x": -32.78125,
+ "pos_y": -75.40625,
+ "pos_z": 53.71875,
+ "stations": "Bunsen Gateway,Gilliland's Folly"
+ },
+ {
+ "name": "Wike Mandji",
+ "pos_x": -116.9375,
+ "pos_y": -105.65625,
+ "pos_z": -10.1875,
+ "stations": "Card Dock,MacLean Settlement,Carrasco Ring,Warner Port,Carleson Bastion"
+ },
+ {
+ "name": "Wikegarak",
+ "pos_x": 112.75,
+ "pos_y": -169.21875,
+ "pos_z": -47.375,
+ "stations": "Shea Station,Alphonsi Orbital,Hansen Survey,Hill Plant"
+ },
+ {
+ "name": "Wikehali",
+ "pos_x": 104.40625,
+ "pos_y": 33.53125,
+ "pos_z": 109.5,
+ "stations": "Pelt Hub,Vonarburg Mines"
+ },
+ {
+ "name": "Wikianji",
+ "pos_x": -124.625,
+ "pos_y": -12.5,
+ "pos_z": 75.6875,
+ "stations": "Junlong Terminal,Thiele Mines"
+ },
+ {
+ "name": "Wikin",
+ "pos_x": -41.875,
+ "pos_y": 14.9375,
+ "pos_z": 125.9375,
+ "stations": "Friend Colony,Ali Hub"
+ },
+ {
+ "name": "Wikini",
+ "pos_x": 139.71875,
+ "pos_y": -191.125,
+ "pos_z": -67.375,
+ "stations": "Anderson Port,Tilman Base"
+ },
+ {
+ "name": "Wikira",
+ "pos_x": -159.6875,
+ "pos_y": -63.03125,
+ "pos_z": 0,
+ "stations": "d'Eyncourt Dock,Forsskal Arsenal,Chebyshev Plant"
+ },
+ {
+ "name": "Wikmeang",
+ "pos_x": -135.71875,
+ "pos_y": 20.8125,
+ "pos_z": -43.0625,
+ "stations": "Henry Ring,Coleman Gateway,Bridges Orbital,Auld Gateway"
+ },
+ {
+ "name": "Wikmeang Mu",
+ "pos_x": -33.96875,
+ "pos_y": 45.34375,
+ "pos_z": -122.1875,
+ "stations": "Mohmand Relay"
+ },
+ {
+ "name": "Wikmeani",
+ "pos_x": 55.84375,
+ "pos_y": 42.75,
+ "pos_z": -157.0625,
+ "stations": "Forbes Oasis,Desargues Oasis,Palmer Holdings"
+ },
+ {
+ "name": "Wikmunda",
+ "pos_x": -83.21875,
+ "pos_y": -43.1875,
+ "pos_z": 25.625,
+ "stations": "Moore Vision"
+ },
+ {
+ "name": "Wikmung",
+ "pos_x": 116.6875,
+ "pos_y": -240.75,
+ "pos_z": 76.3125,
+ "stations": "Vess Terminal,Henry Port,Safdie's Inheritance,Goldschmidt Vision,Auwers Silo"
+ },
+ {
+ "name": "Wikna",
+ "pos_x": -120.78125,
+ "pos_y": 42,
+ "pos_z": 53,
+ "stations": "Elion Terminal,Barsanti Station,Hunziker Orbital,Buffett Orbital,Fraley Orbital,Bramah Station,Mattingly Dock,Tsunenaga Plant,Brunel Palace,Korzun's Folly"
+ },
+ {
+ "name": "Wiknani",
+ "pos_x": 14.4375,
+ "pos_y": -120.28125,
+ "pos_z": -58.03125,
+ "stations": "Fowler Refinery,Steinmuller Works,Messier Colony"
+ },
+ {
+ "name": "Wiknariu",
+ "pos_x": 128.28125,
+ "pos_y": -75.65625,
+ "pos_z": 35.0625,
+ "stations": "Green City,Hoffmeister Keep"
+ },
+ {
+ "name": "Wiknatanja",
+ "pos_x": -3.90625,
+ "pos_y": -0.15625,
+ "pos_z": 95.9375,
+ "stations": "Joliot-Curie Enterprise,Kizim Dock"
+ },
+ {
+ "name": "Wilastsi",
+ "pos_x": -84,
+ "pos_y": -21.84375,
+ "pos_z": -88.28125,
+ "stations": "Thomas Landing"
+ },
+ {
+ "name": "Wilatha Wang",
+ "pos_x": -38.8125,
+ "pos_y": 77.21875,
+ "pos_z": -75.4375,
+ "stations": "Kizim Depot,Zewail Dock"
+ },
+ {
+ "name": "Wilia",
+ "pos_x": -3.5,
+ "pos_y": -54.375,
+ "pos_z": 100.65625,
+ "stations": "Tokarev Settlement,Lounge Enterprise,Hurley Port,Vinogradov Ring"
+ },
+ {
+ "name": "Wiliajuk",
+ "pos_x": 74.6875,
+ "pos_y": 88.78125,
+ "pos_z": -120.9375,
+ "stations": "Boltzmann Ring"
+ },
+ {
+ "name": "Wilingura",
+ "pos_x": 71.6875,
+ "pos_y": 88.8125,
+ "pos_z": 72.90625,
+ "stations": "Brahe Point,Dana Gateway"
+ },
+ {
+ "name": "Wilintae",
+ "pos_x": 140,
+ "pos_y": 40.375,
+ "pos_z": -26.15625,
+ "stations": "Cramer Dock,Isherwood Horizons,Herreshoff Holdings,Pond Works,Spassky Camp"
+ },
+ {
+ "name": "Wiljandiltu",
+ "pos_x": 117.34375,
+ "pos_y": -187.28125,
+ "pos_z": -57.125,
+ "stations": "Royo Camp"
+ },
+ {
+ "name": "Wiljanherbi",
+ "pos_x": 38.5625,
+ "pos_y": 0.6875,
+ "pos_z": 107.6875,
+ "stations": "Bayliss Enterprise,Revin Survey,Kooi Hub,Precourt Station"
+ },
+ {
+ "name": "Willapa",
+ "pos_x": -11.6875,
+ "pos_y": -10.8125,
+ "pos_z": 65.5625,
+ "stations": "Vasquez de Coronado Ring,Harness Orbital,Nordenskiold Observatory,Lagrange Keep"
+ },
+ {
+ "name": "Wille",
+ "pos_x": 34.03125,
+ "pos_y": 11.21875,
+ "pos_z": 106.53125,
+ "stations": "White Base,Forsskal Outpost,Fibonacci Camp"
+ },
+ {
+ "name": "Willionai",
+ "pos_x": -135.5,
+ "pos_y": -77.75,
+ "pos_z": 82.125,
+ "stations": "Yurchikhin Dock"
+ },
+ {
+ "name": "Winagiman",
+ "pos_x": 131.15625,
+ "pos_y": -136,
+ "pos_z": 68.34375,
+ "stations": "Alexandria Base,Louis de Lacaille Mines,Hevelius Works"
+ },
+ {
+ "name": "Winah",
+ "pos_x": 63.90625,
+ "pos_y": -161.15625,
+ "pos_z": -72.5625,
+ "stations": "Amedeo Plana Dock,Gurshtein Orbital,Carpini Holdings,Kratman Beacon,Yuzhe Prospect"
+ },
+ {
+ "name": "Winasak",
+ "pos_x": 142.71875,
+ "pos_y": -13.59375,
+ "pos_z": -23.03125,
+ "stations": "Shunkai Estate,O'Neill Station"
+ },
+ {
+ "name": "Windadones",
+ "pos_x": 147.78125,
+ "pos_y": -73.46875,
+ "pos_z": 119.5,
+ "stations": "Hansen Relay"
+ },
+ {
+ "name": "Windali",
+ "pos_x": -60.25,
+ "pos_y": 98.9375,
+ "pos_z": 41.3125,
+ "stations": "Reynolds Beacon"
+ },
+ {
+ "name": "Windiil",
+ "pos_x": 13.65625,
+ "pos_y": -179,
+ "pos_z": 92.53125,
+ "stations": "Bouwens Vision"
+ },
+ {
+ "name": "Windin",
+ "pos_x": -75.6875,
+ "pos_y": 14.75,
+ "pos_z": -68.3125,
+ "stations": "McKay Hub,Wells Works,Fettman Depot"
+ },
+ {
+ "name": "Windit",
+ "pos_x": 81.5,
+ "pos_y": -79.09375,
+ "pos_z": -23.6875,
+ "stations": "Delsanti Hub,Allen St. John Port"
+ },
+ {
+ "name": "Windizetet",
+ "pos_x": -45.125,
+ "pos_y": 24.0625,
+ "pos_z": -131.3125,
+ "stations": "Paulmier de Gonneville Dock,Norgay Dock,Dyr Dock,Hausdorff Horizons,McDevitt Point"
+ },
+ {
+ "name": "Windjabar",
+ "pos_x": 111.3125,
+ "pos_y": -143.625,
+ "pos_z": -4.4375,
+ "stations": "Xin City"
+ },
+ {
+ "name": "Windjeri",
+ "pos_x": 58.28125,
+ "pos_y": -133.21875,
+ "pos_z": 144.15625,
+ "stations": "Kotelnikov Orbital,Schachner Enterprise,Burstein Bastion"
+ },
+ {
+ "name": "Windjet",
+ "pos_x": 152.25,
+ "pos_y": -95.625,
+ "pos_z": 125.78125,
+ "stations": "Sternbach Port"
+ },
+ {
+ "name": "Windji",
+ "pos_x": 24.9375,
+ "pos_y": -208.9375,
+ "pos_z": 21.53125,
+ "stations": "Kaufmanis Vision,van der Riet Woolley Settlement,Hoshi Holdings"
+ },
+ {
+ "name": "Windri",
+ "pos_x": 129.5625,
+ "pos_y": -0.1875,
+ "pos_z": -41.84375,
+ "stations": "Ashton Colony,Davis Laboratory"
+ },
+ {
+ "name": "Windumlia",
+ "pos_x": 114.0625,
+ "pos_y": -59.5,
+ "pos_z": 77.03125,
+ "stations": "Kiernan Relay,Stevenson Landing,Krenkel Colony"
+ },
+ {
+ "name": "Windumu",
+ "pos_x": -13.46875,
+ "pos_y": 35,
+ "pos_z": -116.90625,
+ "stations": "Howard Works"
+ },
+ {
+ "name": "Winiama",
+ "pos_x": 37.96875,
+ "pos_y": 54.375,
+ "pos_z": 39.625,
+ "stations": "Bowersox Port"
+ },
+ {
+ "name": "Winians",
+ "pos_x": -138.46875,
+ "pos_y": -70.59375,
+ "pos_z": 80.40625,
+ "stations": "Bombelli Relay"
+ },
+ {
+ "name": "Winifex",
+ "pos_x": 101.6875,
+ "pos_y": -119.21875,
+ "pos_z": -60.4375,
+ "stations": "Barbuy Orbital,Gonnessiat City,Dunlop Station,Millosevich Barracks"
+ },
+ {
+ "name": "Wintii",
+ "pos_x": 79.59375,
+ "pos_y": 81.9375,
+ "pos_z": 79,
+ "stations": "Whymper Landing"
+ },
+ {
+ "name": "Wira",
+ "pos_x": 87.34375,
+ "pos_y": -65.0625,
+ "pos_z": -52.3125,
+ "stations": "Hugh Dock"
+ },
+ {
+ "name": "Wiradjuri",
+ "pos_x": 188.6875,
+ "pos_y": -112.1875,
+ "pos_z": 99.125,
+ "stations": "Bok Orbital,Chacornac Orbital,Wood Terminal"
+ },
+ {
+ "name": "Wirang",
+ "pos_x": 46.75,
+ "pos_y": -197.90625,
+ "pos_z": 106.46875,
+ "stations": "Schmidt Vision,Tiptree Survey,Zaschka Platform"
+ },
+ {
+ "name": "Wirangu",
+ "pos_x": -144.75,
+ "pos_y": -17.09375,
+ "pos_z": 62.90625,
+ "stations": "Auld Settlement,Petaja Terminal,Fowler Installation"
+ },
+ {
+ "name": "Wirao",
+ "pos_x": 33.1875,
+ "pos_y": 144.28125,
+ "pos_z": -27.78125,
+ "stations": "Merbold Landing"
+ },
+ {
+ "name": "Wirari",
+ "pos_x": -94.3125,
+ "pos_y": 60.28125,
+ "pos_z": 104.5625,
+ "stations": "Buchli Works,Humboldt Base,Hippalus Prospect"
+ },
+ {
+ "name": "Wirdh",
+ "pos_x": 27.5625,
+ "pos_y": 94.8125,
+ "pos_z": -49.65625,
+ "stations": "Virchow Station,Saberhagen Survey,Merril Terminal,Bartoe Station"
+ },
+ {
+ "name": "Wirdi",
+ "pos_x": 95.375,
+ "pos_y": -119.375,
+ "pos_z": 139.5,
+ "stations": "Druillet Mines"
+ },
+ {
+ "name": "Wirdiani",
+ "pos_x": -114.625,
+ "pos_y": -21.125,
+ "pos_z": 93.25,
+ "stations": "Alenquer Beacon,Vespucci Station,Stafford Depot,O'Brien Vision"
+ },
+ {
+ "name": "Wirdii",
+ "pos_x": 148.59375,
+ "pos_y": -75.125,
+ "pos_z": 85.46875,
+ "stations": "Watson Dock"
+ },
+ {
+ "name": "Wirdisci",
+ "pos_x": 90.5625,
+ "pos_y": -8.0625,
+ "pos_z": -92.4375,
+ "stations": "Coulter Vision,Blair Vista,Barcelos Stop,Krupkat Gateway"
+ },
+ {
+ "name": "Wirian",
+ "pos_x": 41.9375,
+ "pos_y": 69.59375,
+ "pos_z": 149.5625,
+ "stations": "Haller Landing"
+ },
+ {
+ "name": "Wirigans",
+ "pos_x": -125.6875,
+ "pos_y": -10.625,
+ "pos_z": -148.15625,
+ "stations": "Geston Horizons,Maire Colony,Stjepan Seljan Station"
+ },
+ {
+ "name": "Wirnako",
+ "pos_x": -93.46875,
+ "pos_y": -108.625,
+ "pos_z": 6.40625,
+ "stations": "Moffitt Platform,Morgan Installation,von Bellingshausen Lab,Cobb Landing"
+ },
+ {
+ "name": "Wirnga",
+ "pos_x": 67.8125,
+ "pos_y": -220.46875,
+ "pos_z": 64.21875,
+ "stations": "Shoujing Platform"
+ },
+ {
+ "name": "Wirngir",
+ "pos_x": -159.4375,
+ "pos_y": -20.6875,
+ "pos_z": 20.875,
+ "stations": "Korniyenko Colony"
+ },
+ {
+ "name": "Wirnici",
+ "pos_x": 22.75,
+ "pos_y": -129.59375,
+ "pos_z": 70.46875,
+ "stations": "Kulin Dock"
+ },
+ {
+ "name": "Wirnir",
+ "pos_x": 118.96875,
+ "pos_y": -243.4375,
+ "pos_z": 60.0625,
+ "stations": "Dyr Legacy,Kettle Survey"
+ },
+ {
+ "name": "Wisas",
+ "pos_x": 142.09375,
+ "pos_y": -65.25,
+ "pos_z": -89.90625,
+ "stations": "Walker's Claim,Noriega Camp,Roberts Works"
+ },
+ {
+ "name": "WISE 0855-0714",
+ "pos_x": 6.53125,
+ "pos_y": -2.15625,
+ "pos_z": 2.03125,
+ "stations": "Yamazaki Landing"
+ },
+ {
+ "name": "WISE 1506+7027",
+ "pos_x": -7.375,
+ "pos_y": 7.09375,
+ "pos_z": -2.4375,
+ "stations": "Borman Port,Dobrovolskiy Enterprise,Parise Dock,Stafford Penal colony"
+ },
+ {
+ "name": "WISE 1800+0134",
+ "pos_x": -13.40625,
+ "pos_y": 5.96875,
+ "pos_z": 24.65625,
+ "stations": "Underwood Dock,Hopkins' Inheritance,Makarov Silo,Manarov Terminal,Alexandrov Settlement"
+ },
+ {
+ "name": "WISE J000517",
+ "pos_x": -19.46875,
+ "pos_y": -9.59375,
+ "pos_z": -8.25,
+ "stations": "Hackworth Refinery"
+ },
+ {
+ "name": "Wishas",
+ "pos_x": -68.875,
+ "pos_y": -151.4375,
+ "pos_z": -26.78125,
+ "stations": "Potagos Legacy"
+ },
+ {
+ "name": "Wishia",
+ "pos_x": 110.9375,
+ "pos_y": -95.25,
+ "pos_z": 129.8125,
+ "stations": "Baille Settlement,Gaensler Station,Hall City,Willis' Inheritance"
+ },
+ {
+ "name": "Witchhaul",
+ "pos_x": -13.25,
+ "pos_y": -52.0625,
+ "pos_z": -65.875,
+ "stations": "Hornby Terminal,Hawkes' Progress,McMullen Enterprise,Kozlov Base,Boodt Keep"
+ },
+ {
+ "name": "Wito",
+ "pos_x": -91.875,
+ "pos_y": 105.78125,
+ "pos_z": 118.53125,
+ "stations": "Archer Orbital,Baird Colony,Russell Ring"
+ },
+ {
+ "name": "Witoa",
+ "pos_x": 116.125,
+ "pos_y": -63.75,
+ "pos_z": 29.84375,
+ "stations": "Moore Relay,McIntosh Installation,Smoot Hangar"
+ },
+ {
+ "name": "Witola",
+ "pos_x": -120.53125,
+ "pos_y": -0.84375,
+ "pos_z": 18.6875,
+ "stations": "Shull Port"
+ },
+ {
+ "name": "Witolia",
+ "pos_x": -92.875,
+ "pos_y": 37.40625,
+ "pos_z": 85.5,
+ "stations": "Maury Reach,Anthony de la Roche Prospect"
+ },
+ {
+ "name": "Witou",
+ "pos_x": 29.6875,
+ "pos_y": -127.5625,
+ "pos_z": -50.0625,
+ "stations": "Sugano Ring,Malvern Vision,Leopold Heckmann Terminal"
+ },
+ {
+ "name": "Witsanukam",
+ "pos_x": -44.6875,
+ "pos_y": -221.375,
+ "pos_z": 50.125,
+ "stations": "Lane Vision,Polikarpov Gateway,Puiseux City,Nansen Depot,Escobar Lab"
+ },
+ {
+ "name": "Witsegernir",
+ "pos_x": 111.6875,
+ "pos_y": -76.59375,
+ "pos_z": 95.875,
+ "stations": "Extra Gateway,Abell Port,Elson Port"
+ },
+ {
+ "name": "Wiyo",
+ "pos_x": -62.03125,
+ "pos_y": -135.90625,
+ "pos_z": 79.59375,
+ "stations": "Rangarajan Mines"
+ },
+ {
+ "name": "Wiyomisii",
+ "pos_x": 73.875,
+ "pos_y": 10.96875,
+ "pos_z": -102.9375,
+ "stations": "Anvil Ring,Harrison Platform,Bayley Settlement"
+ },
+ {
+ "name": "Wiyotset",
+ "pos_x": -115.65625,
+ "pos_y": -80.25,
+ "pos_z": -63.53125,
+ "stations": "Watts Mines"
+ },
+ {
+ "name": "Wo 9067 B",
+ "pos_x": -31.46875,
+ "pos_y": -102.21875,
+ "pos_z": -65.625,
+ "stations": "Lee Penal colony,Hambly Outpost"
+ },
+ {
+ "name": "Wo 9203",
+ "pos_x": 11,
+ "pos_y": 7.8125,
+ "pos_z": -135.25,
+ "stations": "Krylov Base,Hendel Laboratory"
+ },
+ {
+ "name": "Wo 9251 B",
+ "pos_x": 64.875,
+ "pos_y": 41.65625,
+ "pos_z": -93,
+ "stations": "Jenner Station,Sinclair Enterprise,Shaw Port,Barreiros' Progress"
+ },
+ {
+ "name": "Wo 9512 B",
+ "pos_x": 73.90625,
+ "pos_y": 10.875,
+ "pos_z": 100.75,
+ "stations": "Avicenna Station,Clark Hub"
+ },
+ {
+ "name": "Wo 9812 B",
+ "pos_x": -38.78125,
+ "pos_y": -142.5,
+ "pos_z": 48.1875,
+ "stations": "Chandra Port,Fozard Lab,Tanaka Point"
+ },
+ {
+ "name": "Woda",
+ "pos_x": -55.875,
+ "pos_y": -78.3125,
+ "pos_z": -80.1875,
+ "stations": "Cooper Vision"
+ },
+ {
+ "name": "Wodaabe",
+ "pos_x": 131.875,
+ "pos_y": 31.84375,
+ "pos_z": -42.90625,
+ "stations": "Kubasov Station"
+ },
+ {
+ "name": "Wodavelia",
+ "pos_x": 149.15625,
+ "pos_y": 19.75,
+ "pos_z": 7.8125,
+ "stations": "Maury Oasis,Henson Prospect"
+ },
+ {
+ "name": "Wodiaei",
+ "pos_x": -33.3125,
+ "pos_y": 101.53125,
+ "pos_z": 27.75,
+ "stations": "Alvares Depot,Harness Platform,Lowry Terminal"
+ },
+ {
+ "name": "Wodimui",
+ "pos_x": 27.65625,
+ "pos_y": -67.46875,
+ "pos_z": -13.78125,
+ "stations": "Ryle Settlement,Dixon Dock,Wallis Platform"
+ },
+ {
+ "name": "Wodiwodi",
+ "pos_x": -76.15625,
+ "pos_y": -23.1875,
+ "pos_z": -34.5,
+ "stations": "Polansky City,Worlidge Landing,Hayden Landing,Eyharts Orbital,Edgeworth Station"
+ },
+ {
+ "name": "Wogaiawong",
+ "pos_x": 126.71875,
+ "pos_y": 5.5,
+ "pos_z": -106.71875,
+ "stations": "Jordan Terminal,Anthony de la Roche's Claim,Zajdel Refinery"
+ },
+ {
+ "name": "Wogam",
+ "pos_x": 36.8125,
+ "pos_y": -100.5,
+ "pos_z": -134.125,
+ "stations": "Maddox Gateway,Vardeman City,MacVicar Hub,Musa al-Khwarizmi Works"
+ },
+ {
+ "name": "Wogama",
+ "pos_x": -88.46875,
+ "pos_y": -35.3125,
+ "pos_z": 53.09375,
+ "stations": "Veach Terminal"
+ },
+ {
+ "name": "Wogaman",
+ "pos_x": -0.59375,
+ "pos_y": 0.21875,
+ "pos_z": 134.8125,
+ "stations": "Sellers Hangar,Borisenko Hub"
+ },
+ {
+ "name": "Wolf 10",
+ "pos_x": -71.28125,
+ "pos_y": -3.34375,
+ "pos_z": -43.65625,
+ "stations": "Townshend Orbital,Macquorn Rankine Station"
+ },
+ {
+ "name": "Wolf 1003",
+ "pos_x": -91.875,
+ "pos_y": -76.25,
+ "pos_z": 33.625,
+ "stations": "Pauling Outpost,Thuot Beacon"
+ },
+ {
+ "name": "Wolf 1019",
+ "pos_x": -61.28125,
+ "pos_y": -56.03125,
+ "pos_z": 24.5625,
+ "stations": "MacLean Dock"
+ },
+ {
+ "name": "Wolf 1022",
+ "pos_x": -64.625,
+ "pos_y": -64.15625,
+ "pos_z": 29.125,
+ "stations": "Deluc Ring,McDivitt Port"
+ },
+ {
+ "name": "Wolf 1039",
+ "pos_x": -24.75,
+ "pos_y": -38.28125,
+ "pos_z": 1.375,
+ "stations": "Haise Horizons,Wolf Horizons"
+ },
+ {
+ "name": "Wolf 1042",
+ "pos_x": -88.8125,
+ "pos_y": -128.15625,
+ "pos_z": -8.75,
+ "stations": "Sinclair Port,Grissom Orbital,Parsons Dock,Bethe Landing,Birkhoff Settlement"
+ },
+ {
+ "name": "Wolf 1051",
+ "pos_x": -32.40625,
+ "pos_y": -43.75,
+ "pos_z": -6.875,
+ "stations": "Jemison Horizons"
+ },
+ {
+ "name": "Wolf 1060",
+ "pos_x": 42.25,
+ "pos_y": 58.3125,
+ "pos_z": 30.875,
+ "stations": "Pawelczyk Hangar,Neumann Survey"
+ },
+ {
+ "name": "Wolf 109",
+ "pos_x": -32.03125,
+ "pos_y": -105.125,
+ "pos_z": -69.21875,
+ "stations": "Blaylock Settlement,Rangarajan Station"
+ },
+ {
+ "name": "Wolf 110",
+ "pos_x": -26.875,
+ "pos_y": -79.8125,
+ "pos_z": -55.3125,
+ "stations": "Clute City,Shalatula Gateway,Zamyatin Penal colony,Goldstein Beacon"
+ },
+ {
+ "name": "Wolf 1112",
+ "pos_x": -142,
+ "pos_y": 32.09375,
+ "pos_z": 14.78125,
+ "stations": "Swift Hub,Phillifent Colony,Swigert Lab"
+ },
+ {
+ "name": "Wolf 1148",
+ "pos_x": -87.84375,
+ "pos_y": -31.75,
+ "pos_z": 8.59375,
+ "stations": "Stephenson Gateway,Peterson Port,Bursch Enterprise,Treshchov Station,Avicenna Port,Fontana Station,Vinogradov Ring,Ryman Enterprise,Petaja Barracks,Arnarson Enterprise,Dorsett Station"
+ },
+ {
+ "name": "Wolf 1176",
+ "pos_x": -110.125,
+ "pos_y": -43.84375,
+ "pos_z": 7.4375,
+ "stations": "McMahon Landing,Vela Camp,Hobaugh Colony"
+ },
+ {
+ "name": "Wolf 1182",
+ "pos_x": -71.6875,
+ "pos_y": -20.96875,
+ "pos_z": -0.9375,
+ "stations": "Clifton Hangar,Robson City,Elgin Escape"
+ },
+ {
+ "name": "Wolf 12",
+ "pos_x": -44.65625,
+ "pos_y": -21.53125,
+ "pos_z": -27.1875,
+ "stations": "Bogdanov Vision,Menzies Platform,Kent Base"
+ },
+ {
+ "name": "Wolf 121",
+ "pos_x": -38.90625,
+ "pos_y": -144.59375,
+ "pos_z": -97.4375,
+ "stations": "Levinson Dock,Stackpole Relay,Morgan Dock,Wollheim Landing"
+ },
+ {
+ "name": "Wolf 1230",
+ "pos_x": -137,
+ "pos_y": -47.59375,
+ "pos_z": -5.28125,
+ "stations": "Guidoni Dock,Satcher Dock,Wilson Keep,Lupoff Terminal"
+ },
+ {
+ "name": "Wolf 124",
+ "pos_x": -7.25,
+ "pos_y": -27.15625,
+ "pos_z": -19.09375,
+ "stations": "Willis Port,Byrd Point,Yamazaki Station,Roosa Hub,Jacobi Vision"
+ },
+ {
+ "name": "Wolf 1241",
+ "pos_x": -31.0625,
+ "pos_y": -47.40625,
+ "pos_z": -107.21875,
+ "stations": "Thomas Orbital,Gordon Relay"
+ },
+ {
+ "name": "Wolf 1268",
+ "pos_x": -48.40625,
+ "pos_y": -65.0625,
+ "pos_z": -167.25,
+ "stations": "Williams Base"
+ },
+ {
+ "name": "Wolf 127",
+ "pos_x": -25.53125,
+ "pos_y": -76.1875,
+ "pos_z": -60.28125,
+ "stations": "Chaudhary Terminal,Yeliseyev Ring,Glashow Hub,al-Khayyam Reach"
+ },
+ {
+ "name": "Wolf 1278",
+ "pos_x": -11.3125,
+ "pos_y": -17.65625,
+ "pos_z": -43.3125,
+ "stations": "Scott Settlement,Maler Hub,Alvares Survey"
+ },
+ {
+ "name": "Wolf 1297",
+ "pos_x": -22.375,
+ "pos_y": -44.125,
+ "pos_z": -104.25,
+ "stations": "Sacco Base,Szebehely Point,Ivins Depot"
+ },
+ {
+ "name": "Wolf 1301",
+ "pos_x": -15.4375,
+ "pos_y": -34.6875,
+ "pos_z": -79.40625,
+ "stations": "Saunders's Dive,Denton Installation,Menezes Installation,Herreshoff Lab,Baxter Base"
+ },
+ {
+ "name": "Wolf 1316",
+ "pos_x": -19.375,
+ "pos_y": -44.59375,
+ "pos_z": -108.0625,
+ "stations": "Lerman Port"
+ },
+ {
+ "name": "Wolf 1323",
+ "pos_x": -22.125,
+ "pos_y": -17.625,
+ "pos_z": -38.375,
+ "stations": "DENIS FILIPPOV,Rattus High"
+ },
+ {
+ "name": "Wolf 1326",
+ "pos_x": -71.46875,
+ "pos_y": -82.71875,
+ "pos_z": 50.25,
+ "stations": "Frimout Terminal,Gaspar de Portola Vista,Thurston Point"
+ },
+ {
+ "name": "Wolf 1329",
+ "pos_x": -17.3125,
+ "pos_y": -21.28125,
+ "pos_z": 11.34375,
+ "stations": "Leestma Keep,Vesalius Settlement,Wellman City"
+ },
+ {
+ "name": "Wolf 1341",
+ "pos_x": -87.21875,
+ "pos_y": -100.9375,
+ "pos_z": 58.125,
+ "stations": "Clark Platform,Cramer Keep"
+ },
+ {
+ "name": "Wolf 1346",
+ "pos_x": -46.3125,
+ "pos_y": -8.21875,
+ "pos_z": 19,
+ "stations": "Hawkes Landing,Kovalevskaya Refinery,Arber's Progress,Vonarburg Plant"
+ },
+ {
+ "name": "Wolf 1373",
+ "pos_x": -40.4375,
+ "pos_y": -11.75,
+ "pos_z": 15.71875,
+ "stations": "Allen Refinery,Alas Landing,Libby Hub,Fu Prospect,Springer Installation"
+ },
+ {
+ "name": "Wolf 1398",
+ "pos_x": -123.125,
+ "pos_y": 67.9375,
+ "pos_z": 29.75,
+ "stations": "Surayev Stop,Apgar Installation"
+ },
+ {
+ "name": "Wolf 1409",
+ "pos_x": -56.125,
+ "pos_y": 29.8125,
+ "pos_z": 9.0625,
+ "stations": "Hyecho's Claim,Walheim Horizons,Zamka Terminal,Kroehl Base"
+ },
+ {
+ "name": "Wolf 1414",
+ "pos_x": -76.46875,
+ "pos_y": 39.03125,
+ "pos_z": 16.4375,
+ "stations": "Baker Terminal,Robinson Port,Pond Depot"
+ },
+ {
+ "name": "Wolf 1415",
+ "pos_x": -144.25,
+ "pos_y": 75.375,
+ "pos_z": 18.75,
+ "stations": "Barba Settlement,Malocello Depot"
+ },
+ {
+ "name": "Wolf 1431",
+ "pos_x": 40.3125,
+ "pos_y": 129.34375,
+ "pos_z": -2.1875,
+ "stations": "Qureshi Enterprise,Rey Installation"
+ },
+ {
+ "name": "Wolf 1434",
+ "pos_x": 27.09375,
+ "pos_y": 133.15625,
+ "pos_z": -8.875,
+ "stations": "Ockels Horizons,Kadenyuk Survey,Goeschke Orbital"
+ },
+ {
+ "name": "Wolf 1437",
+ "pos_x": 35.71875,
+ "pos_y": 106.5,
+ "pos_z": 2.71875,
+ "stations": "Sutcliffe Enterprise,Schroeder Terminal,Ford Installation"
+ },
+ {
+ "name": "Wolf 1438",
+ "pos_x": 21.21875,
+ "pos_y": 88.90625,
+ "pos_z": -2.40625,
+ "stations": "Shaw Station"
+ },
+ {
+ "name": "Wolf 1449",
+ "pos_x": 17.96875,
+ "pos_y": -14.15625,
+ "pos_z": -35.8125,
+ "stations": "Behring Terminal"
+ },
+ {
+ "name": "Wolf 1453",
+ "pos_x": 7.96875,
+ "pos_y": -6,
+ "pos_z": -15.53125,
+ "stations": "Wagner Station,Cartier City,Byrd City,Lerman Dock,Akers Gateway,Godel Terminal"
+ },
+ {
+ "name": "Wolf 1458",
+ "pos_x": 75.84375,
+ "pos_y": -49.125,
+ "pos_z": -134.125,
+ "stations": "Townshend Vision,Linenger Enterprise"
+ },
+ {
+ "name": "Wolf 1463",
+ "pos_x": -74.09375,
+ "pos_y": 0.75,
+ "pos_z": 158.1875,
+ "stations": "Young Dock"
+ },
+ {
+ "name": "Wolf 1481",
+ "pos_x": 5.1875,
+ "pos_y": 13.375,
+ "pos_z": 13.5625,
+ "stations": "Velazquez Gateway,Jones Orbital,Seddon Station,Levinson Arsenal"
+ },
+ {
+ "name": "Wolf 1487",
+ "pos_x": 14.5625,
+ "pos_y": 62.9375,
+ "pos_z": 25.71875,
+ "stations": "d'Eyncourt City,Steinmuller Outpost"
+ },
+ {
+ "name": "Wolf 1509",
+ "pos_x": -35.8125,
+ "pos_y": 3.84375,
+ "pos_z": 38.21875,
+ "stations": "Beltrami's Claim,Bolotov Dock,MacLean Port"
+ },
+ {
+ "name": "Wolf 1515",
+ "pos_x": -49.75,
+ "pos_y": -64.84375,
+ "pos_z": -41.25,
+ "stations": "WotNoName Station"
+ },
+ {
+ "name": "Wolf 152",
+ "pos_x": -41.125,
+ "pos_y": -15.125,
+ "pos_z": -71.78125,
+ "stations": "Julian Reach"
+ },
+ {
+ "name": "Wolf 1549",
+ "pos_x": -83.6875,
+ "pos_y": -148.03125,
+ "pos_z": 90.3125,
+ "stations": "Clairaut Prospect"
+ },
+ {
+ "name": "Wolf 186",
+ "pos_x": -55.1875,
+ "pos_y": -14.65625,
+ "pos_z": -96.5625,
+ "stations": "Conrad Gateway,Sharman Port,Kuhn Station,Salk Plant"
+ },
+ {
+ "name": "Wolf 202",
+ "pos_x": -70.15625,
+ "pos_y": -27.59375,
+ "pos_z": -140.21875,
+ "stations": "Kozin Market"
+ },
+ {
+ "name": "Wolf 217",
+ "pos_x": -61.125,
+ "pos_y": -22.75,
+ "pos_z": -125.375,
+ "stations": "Barnes Hub,Kippax Outpost,Crook Holdings"
+ },
+ {
+ "name": "Wolf 225",
+ "pos_x": -33,
+ "pos_y": -13.625,
+ "pos_z": -71.5,
+ "stations": "Grandin Landing,Skvortsov Terminal,Shaver Base,Edwards Port"
+ },
+ {
+ "name": "Wolf 229",
+ "pos_x": 2.5625,
+ "pos_y": -26.0625,
+ "pos_z": -106.09375,
+ "stations": "Ford Point,Moon Terminal"
+ },
+ {
+ "name": "Wolf 232",
+ "pos_x": 7.84375,
+ "pos_y": -21.09375,
+ "pos_z": -111.75,
+ "stations": "Savinykh Terminal,Bursch City,Vinci Station,Eskridge Point,Bolger Installation"
+ },
+ {
+ "name": "Wolf 248",
+ "pos_x": 2.78125,
+ "pos_y": 60.3125,
+ "pos_z": -0.4375,
+ "stations": "Hencke Centre,Kaku Hub"
+ },
+ {
+ "name": "Wolf 25",
+ "pos_x": -11.0625,
+ "pos_y": -20.46875,
+ "pos_z": -7.125,
+ "stations": "Anning Base,Bonkers,Minne Orbital,Clement Vista"
+ },
+ {
+ "name": "Wolf 258",
+ "pos_x": 15.40625,
+ "pos_y": -0.78125,
+ "pos_z": -98.28125,
+ "stations": "Frimout Holdings,Constantine Depot"
+ },
+ {
+ "name": "Wolf 265",
+ "pos_x": 10.4375,
+ "pos_y": 7.34375,
+ "pos_z": -128,
+ "stations": "Felice Prospect"
+ },
+ {
+ "name": "Wolf 279",
+ "pos_x": -33.6875,
+ "pos_y": 39.5,
+ "pos_z": -147.96875,
+ "stations": "Bresnik Settlement,Taylor Horizons"
+ },
+ {
+ "name": "Wolf 280",
+ "pos_x": -24.5625,
+ "pos_y": 32.28125,
+ "pos_z": -131.03125,
+ "stations": "Grechko Oasis,Tito Colony,den Berg Depot"
+ },
+ {
+ "name": "Wolf 281",
+ "pos_x": 41.4375,
+ "pos_y": 10.71875,
+ "pos_z": -154.96875,
+ "stations": "Hausdorff Holdings,Narbeth Legacy,Berezin Legacy"
+ },
+ {
+ "name": "Wolf 286",
+ "pos_x": 55.5625,
+ "pos_y": 10.96875,
+ "pos_z": -160.15625,
+ "stations": "Cook Camp,Clark Laboratory"
+ },
+ {
+ "name": "Wolf 287",
+ "pos_x": 8.71875,
+ "pos_y": 3,
+ "pos_z": -30.4375,
+ "stations": "Boas Terminal"
+ },
+ {
+ "name": "Wolf 289",
+ "pos_x": 24.75,
+ "pos_y": 7.0625,
+ "pos_z": -77.375,
+ "stations": "Julian Relay,Kotov Refinery"
+ },
+ {
+ "name": "Wolf 294",
+ "pos_x": 1,
+ "pos_y": 4.9375,
+ "pos_z": -17.53125,
+ "stations": "Stephenson Dock"
+ },
+ {
+ "name": "Wolf 315",
+ "pos_x": -0.34375,
+ "pos_y": 56.03125,
+ "pos_z": -74.125,
+ "stations": "Russ Platform,Cousteau Asylum"
+ },
+ {
+ "name": "Wolf 318",
+ "pos_x": -2.65625,
+ "pos_y": 63.84375,
+ "pos_z": -81.25,
+ "stations": "Gidzenko Enterprise,Karl Diesel Hub,Grover Dock,Manning Lab,Rolland Point"
+ },
+ {
+ "name": "Wolf 324",
+ "pos_x": 24.71875,
+ "pos_y": 41.375,
+ "pos_z": -48.21875,
+ "stations": "Daimler Base,Polya Camp,Weber Port,Lichtenberg Terminal,Bella Gateway"
+ },
+ {
+ "name": "Wolf 325",
+ "pos_x": 36.03125,
+ "pos_y": 63.90625,
+ "pos_z": -72.96875,
+ "stations": "Vasyutin City,Belyanin Platform,Walz Station,Chaudhary Orbital,Teng-hui Dock,Lubin Orbital,Potter City,Grothendieck Settlement,Liska Arsenal,Gresley Survey,Hovell Forum"
+ },
+ {
+ "name": "Wolf 331",
+ "pos_x": 18.28125,
+ "pos_y": 88.1875,
+ "pos_z": -65.21875,
+ "stations": "Tuan Platform,Spedding Relay"
+ },
+ {
+ "name": "Wolf 333",
+ "pos_x": 15.375,
+ "pos_y": 108.875,
+ "pos_z": -81.40625,
+ "stations": "Crown Terminal,Olivas Dock,Alexandria Ring,Byrd Works"
+ },
+ {
+ "name": "Wolf 335",
+ "pos_x": 13.3125,
+ "pos_y": 70.125,
+ "pos_z": -50.3125,
+ "stations": "Blaha Hub,Foale Ring,Miletus Prospect,Culpeper Port,Oleskiw Port,Wilson Hub,Zhigang City,Adams Station,Weyn Mines,Vance Silo,Vance Gateway,Panshin Hub"
+ },
+ {
+ "name": "Wolf 342",
+ "pos_x": 6.78125,
+ "pos_y": 119.40625,
+ "pos_z": -84.40625,
+ "stations": "Leichhardt Plant,Lopez de Villalobos Plant"
+ },
+ {
+ "name": "Wolf 359",
+ "pos_x": 3.875,
+ "pos_y": 6.46875,
+ "pos_z": -1.90625,
+ "stations": "Powell High,Lomas Orbiter,Cayley Enterprise,Tryggvason Installation"
+ },
+ {
+ "name": "Wolf 363",
+ "pos_x": 53.46875,
+ "pos_y": 80.6875,
+ "pos_z": -14.25,
+ "stations": "Pirsan Station,Stith Base,Eisele Station,Moresby Beacon"
+ },
+ {
+ "name": "Wolf 366",
+ "pos_x": 95,
+ "pos_y": 128.75,
+ "pos_z": -16.875,
+ "stations": "Khan Ring"
+ },
+ {
+ "name": "Wolf 369",
+ "pos_x": 30.625,
+ "pos_y": 50.375,
+ "pos_z": -8.875,
+ "stations": "Nicollet Terminal,Howe Platform,den Berg Settlement,Ponce de Leon Enterprise"
+ },
+ {
+ "name": "Wolf 392",
+ "pos_x": 67.21875,
+ "pos_y": 147.84375,
+ "pos_z": -24.25,
+ "stations": "Sullivan Orbital,Gurragchaa Station,Landsteiner Point"
+ },
+ {
+ "name": "Wolf 393",
+ "pos_x": 29.65625,
+ "pos_y": 49.4375,
+ "pos_z": -4.9375,
+ "stations": "Massimino Settlement"
+ },
+ {
+ "name": "Wolf 397",
+ "pos_x": 40,
+ "pos_y": 79.21875,
+ "pos_z": -10.40625,
+ "stations": "Chelbin Service Station,Trophy Camp,Cregglezone,du Fresne Exchange"
+ },
+ {
+ "name": "Wolf 406",
+ "pos_x": 41.0625,
+ "pos_y": 74.125,
+ "pos_z": 8.75,
+ "stations": "Hamilton Gateway,Zillig City,Rothfuss Landing"
+ },
+ {
+ "name": "Wolf 411",
+ "pos_x": 4.875,
+ "pos_y": 111.375,
+ "pos_z": -9.90625,
+ "stations": "Janes Port,Watt-Evans Installation,Samuda's Claim"
+ },
+ {
+ "name": "Wolf 412",
+ "pos_x": 4.40625,
+ "pos_y": 88.40625,
+ "pos_z": -6.09375,
+ "stations": "Borisenko Landing,Low Dock,Bradbury's Folly"
+ },
+ {
+ "name": "Wolf 424",
+ "pos_x": 4.21875,
+ "pos_y": 13.28125,
+ "pos_z": 1.4375,
+ "stations": "Pontes Terminal,Readdy Gateway,Webb Holdings,Alexandrov Base"
+ },
+ {
+ "name": "Wolf 425",
+ "pos_x": 12.5,
+ "pos_y": 146.75,
+ "pos_z": -2.71875,
+ "stations": "Linnehan Orbital,von Helmont Platform,Brooks Base"
+ },
+ {
+ "name": "Wolf 433",
+ "pos_x": 11.71875,
+ "pos_y": 45.0625,
+ "pos_z": 5.1875,
+ "stations": "Ellis Orbital,Romanenko Port"
+ },
+ {
+ "name": "Wolf 437",
+ "pos_x": 7,
+ "pos_y": 26,
+ "pos_z": 4.375,
+ "stations": "Bunsen Platform,Virts Survey"
+ },
+ {
+ "name": "Wolf 440",
+ "pos_x": 39.125,
+ "pos_y": 95.28125,
+ "pos_z": 25.8125,
+ "stations": "Scortia Refinery,Thurston Bastion"
+ },
+ {
+ "name": "Wolf 46",
+ "pos_x": -27.03125,
+ "pos_y": -0.125,
+ "pos_z": -18.625,
+ "stations": "Franklin Dock,Grabe Horizons,Fischer Station"
+ },
+ {
+ "name": "Wolf 476",
+ "pos_x": -64.78125,
+ "pos_y": 120.15625,
+ "pos_z": -34.34375,
+ "stations": "Chadwick Dock,Mitchell Hub"
+ },
+ {
+ "name": "Wolf 485a",
+ "pos_x": 22.90625,
+ "pos_y": 46.71875,
+ "pos_z": 27.28125,
+ "stations": "Faraday Ring,Hoard Gateway"
+ },
+ {
+ "name": "Wolf 490",
+ "pos_x": 19.46875,
+ "pos_y": 66.53125,
+ "pos_z": 33.6875,
+ "stations": "Engle Port,Al-Farabi Depot,Hedley Port,Patsayev Platform,Stokes Works"
+ },
+ {
+ "name": "Wolf 497",
+ "pos_x": 0.125,
+ "pos_y": 41.46875,
+ "pos_z": 11.96875,
+ "stations": "Buffett Vista,Smeaton Vision,Vetulani Holdings,Celsius Observatory,Steinmuller Prospect"
+ },
+ {
+ "name": "Wolf 499",
+ "pos_x": 25.96875,
+ "pos_y": 90.84375,
+ "pos_z": 48.15625,
+ "stations": "Redi Terminal,Crown Beacon,Doi Penal colony"
+ },
+ {
+ "name": "Wolf 504",
+ "pos_x": 15.3125,
+ "pos_y": 90.78125,
+ "pos_z": 39.96875,
+ "stations": "Pinto Enterprise"
+ },
+ {
+ "name": "Wolf 510",
+ "pos_x": 4.21875,
+ "pos_y": 130.6875,
+ "pos_z": 44.5,
+ "stations": "Lewis Vision,Hoffman Installation"
+ },
+ {
+ "name": "Wolf 511",
+ "pos_x": 3.65625,
+ "pos_y": 146.875,
+ "pos_z": 49.21875,
+ "stations": "Buchli Depot,Vizcaino Point"
+ },
+ {
+ "name": "Wolf 517",
+ "pos_x": -6.125,
+ "pos_y": 98.625,
+ "pos_z": 28.34375,
+ "stations": "O'Donnell's Folly,Compton Installation,Gibson Terminal,Litke Plant"
+ },
+ {
+ "name": "Wolf 529",
+ "pos_x": -14.59375,
+ "pos_y": 166.8125,
+ "pos_z": 51.96875,
+ "stations": "Dutton Hub,Laplace Ring,Skvortsov Enterprise,Macomb Barracks,Slusser Terminal"
+ },
+ {
+ "name": "Wolf 54",
+ "pos_x": -39.90625,
+ "pos_y": 1.1875,
+ "pos_z": -27.9375,
+ "stations": "Meeking's Way,Souper Matt,Weber Hangar,Quick Installation,Nicollier Installation"
+ },
+ {
+ "name": "Wolf 555",
+ "pos_x": 4.53125,
+ "pos_y": 64.375,
+ "pos_z": 53.15625,
+ "stations": "Crippen Station,Kavandi Dock,Planck Enterprise,Buchli Station,Jacquard Hub,Gordon Colony,Jahn Enterprise,Glashow Terminal"
+ },
+ {
+ "name": "Wolf 560",
+ "pos_x": 1.28125,
+ "pos_y": 117.21875,
+ "pos_z": 94.125,
+ "stations": "Szebehely Port,Pontes Colony,Person Bastion"
+ },
+ {
+ "name": "Wolf 561",
+ "pos_x": 3.375,
+ "pos_y": 60.21875,
+ "pos_z": 64.125,
+ "stations": "Ramsay City,Lewitt Installation,Garan Terminal"
+ },
+ {
+ "name": "Wolf 562",
+ "pos_x": 1.46875,
+ "pos_y": 12.84375,
+ "pos_z": 15.5625,
+ "stations": "Berners-Lee Terminal,Hopkins Port,King's Inheritance,Nagel Enterprise,Harrison Installation,Faris Gateway"
+ },
+ {
+ "name": "Wolf 573",
+ "pos_x": -6.25,
+ "pos_y": 31.59375,
+ "pos_z": 35.3125,
+ "stations": "Gidzenko Ring"
+ },
+ {
+ "name": "Wolf 579",
+ "pos_x": -29.375,
+ "pos_y": 100.3125,
+ "pos_z": 101.71875,
+ "stations": "Markov's Pride,Gallun's Pride,Hennepin Reach"
+ },
+ {
+ "name": "Wolf 582",
+ "pos_x": -9.0625,
+ "pos_y": 44.59375,
+ "pos_z": 52.4375,
+ "stations": "Lopez-Alegria Port,Sharipov Arsenal"
+ },
+ {
+ "name": "Wolf 587",
+ "pos_x": -7.15625,
+ "pos_y": 37.28125,
+ "pos_z": 44.15625,
+ "stations": "Pytheas' Folly,Reamy City,Cooper Keep"
+ },
+ {
+ "name": "Wolf 620",
+ "pos_x": -40.21875,
+ "pos_y": 63.84375,
+ "pos_z": 40.3125,
+ "stations": "Gerlache Dock,Clairaut Enterprise,Leiber Installation"
+ },
+ {
+ "name": "Wolf 629",
+ "pos_x": -4.0625,
+ "pos_y": 7.6875,
+ "pos_z": 20.09375,
+ "stations": "Gillekens Refinery,Grunsfeld Plant"
+ },
+ {
+ "name": "Wolf 631",
+ "pos_x": -78,
+ "pos_y": 68.53125,
+ "pos_z": 47.5625,
+ "stations": "Szebehely Orbital,Braun Gateway"
+ },
+ {
+ "name": "Wolf 636",
+ "pos_x": -8.75,
+ "pos_y": 11.90625,
+ "pos_z": 31.125,
+ "stations": "Boulton Orbital,Nikolayev Dock,Peterson Relay"
+ },
+ {
+ "name": "Wolf 64",
+ "pos_x": -48.59375,
+ "pos_y": -80.03125,
+ "pos_z": -50.5,
+ "stations": "Massimino Mines,Akiyama Landing,Camarda Beacon"
+ },
+ {
+ "name": "Wolf 65",
+ "pos_x": -68.25,
+ "pos_y": -116.5625,
+ "pos_z": -72.65625,
+ "stations": "Pangborn Port,Savorgnan de Brazza Dock"
+ },
+ {
+ "name": "Wolf 667",
+ "pos_x": -63.34375,
+ "pos_y": 59.03125,
+ "pos_z": 130.625,
+ "stations": "Lorenz Ring,Van Scyoc Relay,Maire Keep"
+ },
+ {
+ "name": "Wolf 687",
+ "pos_x": -44.96875,
+ "pos_y": 40.71875,
+ "pos_z": 101.8125,
+ "stations": "Meitner Hangar"
+ },
+ {
+ "name": "Wolf 718",
+ "pos_x": -10.03125,
+ "pos_y": 8.3125,
+ "pos_z": 21.4375,
+ "stations": "Filipchenko Dock,Song Refinery,White Station,Bloch Plant,Lounge Barracks"
+ },
+ {
+ "name": "Wolf 750",
+ "pos_x": -52.09375,
+ "pos_y": 36.59375,
+ "pos_z": 27.65625,
+ "stations": "Gregory Orbital,Nikolayev Settlement,Neumann Gateway"
+ },
+ {
+ "name": "Wolf 751",
+ "pos_x": -14.78125,
+ "pos_y": 11.09375,
+ "pos_z": 26.78125,
+ "stations": "Reightler Settlement,al-Din Works"
+ },
+ {
+ "name": "Wolf 772",
+ "pos_x": -86.34375,
+ "pos_y": 44.28125,
+ "pos_z": 88.4375,
+ "stations": "LeConte Depot,Hobaugh Platform,Jun Settlement"
+ },
+ {
+ "name": "Wolf 827",
+ "pos_x": -119.6875,
+ "pos_y": 51.1875,
+ "pos_z": 95.25,
+ "stations": "Gresley Ring,Creighton Ring,Fiennes Barracks,Curbeam Horizons,Townshend Observatory"
+ },
+ {
+ "name": "Wolf 865",
+ "pos_x": -50.46875,
+ "pos_y": -9.0625,
+ "pos_z": 34.65625,
+ "stations": "McCulley Ring,Burnell Terminal,Nusslein-Volhard Hub,Leestma Observatory,Yolen Penal colony"
+ },
+ {
+ "name": "Wolf 867",
+ "pos_x": -45.375,
+ "pos_y": -4.09375,
+ "pos_z": 23.84375,
+ "stations": "Dana Terminal,Harbaugh Gateway"
+ },
+ {
+ "name": "Wolf 871",
+ "pos_x": -64.21875,
+ "pos_y": -12.34375,
+ "pos_z": 41,
+ "stations": "Blaha Landing,Al Saud Survey"
+ },
+ {
+ "name": "Wolf 883",
+ "pos_x": -92.125,
+ "pos_y": -66.8125,
+ "pos_z": 87.71875,
+ "stations": "Lorrah's Claim,Flint Hangar,Budrys Terminal"
+ },
+ {
+ "name": "Wolf 896",
+ "pos_x": -26,
+ "pos_y": -27.25,
+ "pos_z": 32.8125,
+ "stations": "Thompson Co-operative,Shaw City,Fraser Enterprise,Hope Laboratory,Hartog Base"
+ },
+ {
+ "name": "Wolf 903",
+ "pos_x": -100.21875,
+ "pos_y": -69.25,
+ "pos_z": 82.75,
+ "stations": "Jahn Port,Mullane Station,Schweickart Terminal,Hausdorff Holdings,Bulgakov Keep"
+ },
+ {
+ "name": "Wolf 906",
+ "pos_x": -27.28125,
+ "pos_y": -25.09375,
+ "pos_z": 28.78125,
+ "stations": "Herbert Dock,Rice Hub"
+ },
+ {
+ "name": "Wolf 914",
+ "pos_x": -119.40625,
+ "pos_y": -79.375,
+ "pos_z": 89.96875,
+ "stations": "Selous Terminal,Hoshi Terminal,Larbalestier Platform,Przhevalsky Keep"
+ },
+ {
+ "name": "Wolf 918",
+ "pos_x": -19,
+ "pos_y": -23.90625,
+ "pos_z": 25.34375,
+ "stations": "Bokeili Vision,Moore Settlement,Frimout Works"
+ },
+ {
+ "name": "Wolf 926",
+ "pos_x": -58.15625,
+ "pos_y": -0.3125,
+ "pos_z": -5.3125,
+ "stations": "Deluc Platform,Eddington Installation"
+ },
+ {
+ "name": "Wolf 953",
+ "pos_x": -50.28125,
+ "pos_y": -38.6875,
+ "pos_z": 21.03125,
+ "stations": "Constantine Settlement,Pellegrino Mines"
+ },
+ {
+ "name": "Wolf 959",
+ "pos_x": -154.78125,
+ "pos_y": -8.5625,
+ "pos_z": -20.59375,
+ "stations": "Morrow Port,Sharp Relay"
+ },
+ {
+ "name": "Wolf 980",
+ "pos_x": -110.9375,
+ "pos_y": -95.3125,
+ "pos_z": 48.03125,
+ "stations": "Al-Kashi Plant,Godwin Settlement"
+ },
+ {
+ "name": "Wolfberg",
+ "pos_x": 50.03125,
+ "pos_y": -38.1875,
+ "pos_z": 106.71875,
+ "stations": "Dogmaa,Litke Mines"
+ },
+ {
+ "name": "Wolonir",
+ "pos_x": 69.90625,
+ "pos_y": 51.25,
+ "pos_z": -138.59375,
+ "stations": "Fisher Hub,Sargent Depot"
+ },
+ {
+ "name": "Woloniugo",
+ "pos_x": 57.78125,
+ "pos_y": 72.25,
+ "pos_z": -68.90625,
+ "stations": "RenenBellot Market,Converse Terminal,Hinz Station"
+ },
+ {
+ "name": "Wondha Mu",
+ "pos_x": 47.65625,
+ "pos_y": -33.96875,
+ "pos_z": 135.71875,
+ "stations": "Killough Terminal"
+ },
+ {
+ "name": "Wondjina",
+ "pos_x": -80.25,
+ "pos_y": 150.6875,
+ "pos_z": 63.625,
+ "stations": "Keyes Station,Hassanein Stop,Fanning Hub,Gelfand Beacon"
+ },
+ {
+ "name": "Wong Guin",
+ "pos_x": 60.0625,
+ "pos_y": -93.96875,
+ "pos_z": -22.96875,
+ "stations": "Heiles Terminal,Mattingly Vision"
+ },
+ {
+ "name": "Wong Mu",
+ "pos_x": 152.21875,
+ "pos_y": -67.875,
+ "pos_z": -77.875,
+ "stations": "Hampson's Pride"
+ },
+ {
+ "name": "Wong Sher",
+ "pos_x": 16.46875,
+ "pos_y": 4.0625,
+ "pos_z": -60.5,
+ "stations": "Lawrence Holdings,Zudov City"
+ },
+ {
+ "name": "Wong Ten",
+ "pos_x": 41.34375,
+ "pos_y": 13.28125,
+ "pos_z": -9.6875,
+ "stations": "Hooke Platform,Garden Laboratory"
+ },
+ {
+ "name": "Wong Zhi",
+ "pos_x": -102.59375,
+ "pos_y": -54.15625,
+ "pos_z": -71.46875,
+ "stations": "Linteris Holdings,Gordon Orbital,Cantor Relay"
+ },
+ {
+ "name": "Wonga",
+ "pos_x": 113,
+ "pos_y": -247.4375,
+ "pos_z": 89.8125,
+ "stations": "Espenak Prospect,Brundage Terminal"
+ },
+ {
+ "name": "Wongaibon",
+ "pos_x": 31.5625,
+ "pos_y": -118.34375,
+ "pos_z": -72.90625,
+ "stations": "Arzachel Landing"
+ },
+ {
+ "name": "Wongarateri",
+ "pos_x": -110.96875,
+ "pos_y": -56.84375,
+ "pos_z": -82.0625,
+ "stations": "Lopez de Villalobos Station,Heaviside Survey,Drzewiecki Dock"
+ },
+ {
+ "name": "Wonggaibuh",
+ "pos_x": 162.1875,
+ "pos_y": 41.21875,
+ "pos_z": -8.90625,
+ "stations": "Revin Landing,Leibniz Hangar"
+ },
+ {
+ "name": "Wonghus",
+ "pos_x": 178.0625,
+ "pos_y": -155.65625,
+ "pos_z": 67.84375,
+ "stations": "Vuia Platform"
+ },
+ {
+ "name": "Wongi",
+ "pos_x": 64.15625,
+ "pos_y": -12.28125,
+ "pos_z": 98.34375,
+ "stations": "Barreiros Survey,Wisniewski-Snerg Enterprise,Schmitt Enterprise,Pogue Ring"
+ },
+ {
+ "name": "Wongkal",
+ "pos_x": -32.78125,
+ "pos_y": -135.40625,
+ "pos_z": 87.71875,
+ "stations": "Narvaez's Claim,Medupe Dock,Austin Base"
+ },
+ {
+ "name": "Wongkamala",
+ "pos_x": 65.84375,
+ "pos_y": -98.40625,
+ "pos_z": 36.84375,
+ "stations": "Bulgarin's Progress,Stromgren Mines"
+ },
+ {
+ "name": "Wongkho",
+ "pos_x": 31.21875,
+ "pos_y": -107.625,
+ "pos_z": 14.28125,
+ "stations": "Hariot Station,Charnas Survey,Flagg Landing,Herndon Survey,Shute Colony"
+ },
+ {
+ "name": "Wongo",
+ "pos_x": -129.90625,
+ "pos_y": -61.5,
+ "pos_z": 74,
+ "stations": "Laplace Prospect"
+ },
+ {
+ "name": "Wongorora",
+ "pos_x": 165.90625,
+ "pos_y": -123.0625,
+ "pos_z": 32.34375,
+ "stations": "Matthews Horizons,Nicolet Base,Harding Orbital,Wild Station"
+ },
+ {
+ "name": "Wongsa",
+ "pos_x": 109.28125,
+ "pos_y": -190.3125,
+ "pos_z": -15.09375,
+ "stations": "Seitter Base,Bode Dock"
+ },
+ {
+ "name": "Wongsun",
+ "pos_x": -50.03125,
+ "pos_y": -40.6875,
+ "pos_z": 151.125,
+ "stations": "Veach Vision,Korzun Orbital,Seddon Hub,Linaweaver Palace"
+ },
+ {
+ "name": "Wongxian Yi",
+ "pos_x": -62.125,
+ "pos_y": -123.125,
+ "pos_z": 14.90625,
+ "stations": "Navigator Outpost,Paez Settlement,Haldeman Camp,Rey Depot"
+ },
+ {
+ "name": "Wongzi",
+ "pos_x": 153.9375,
+ "pos_y": 64.40625,
+ "pos_z": -2.125,
+ "stations": "Hennepin Colony,Webb Survey,Poincare Port,Volterra Forum"
+ },
+ {
+ "name": "Wonne",
+ "pos_x": -136.625,
+ "pos_y": 66.8125,
+ "pos_z": 69.34375,
+ "stations": "Budrys Outpost,Hottot Base"
+ },
+ {
+ "name": "Wonneriti",
+ "pos_x": -92.75,
+ "pos_y": -13.53125,
+ "pos_z": 42.28125,
+ "stations": "Coulomb Terminal,Kettle Prospect,Minkowski Plant,Aldrin Enterprise,Hopkins Port"
+ },
+ {
+ "name": "Wonno",
+ "pos_x": 141.8125,
+ "pos_y": -66.40625,
+ "pos_z": -10.875,
+ "stations": "Brashear City,Nagata Station,Roe Dock"
+ },
+ {
+ "name": "Wonomi",
+ "pos_x": 119.46875,
+ "pos_y": -138.34375,
+ "pos_z": 52.84375,
+ "stations": "Yuzhe Survey,McDermott Station"
+ },
+ {
+ "name": "Wonorna",
+ "pos_x": -89.09375,
+ "pos_y": 118.09375,
+ "pos_z": 44.0625,
+ "stations": "Rotsler Relay,Brule Beacon,Hawkes Prospect"
+ },
+ {
+ "name": "Wonorne Nu",
+ "pos_x": -69.46875,
+ "pos_y": -47,
+ "pos_z": -50.03125,
+ "stations": "Nowak Hub,Bernoulli Terminal,Penn Depot"
+ },
+ {
+ "name": "Wonoto",
+ "pos_x": -122.4375,
+ "pos_y": 89.40625,
+ "pos_z": 70.59375,
+ "stations": "Baird Horizons"
+ },
+ {
+ "name": "Wooptet",
+ "pos_x": -140.59375,
+ "pos_y": 3,
+ "pos_z": 38.09375,
+ "stations": "Korniyenko Gateway,Potagos Base"
+ },
+ {
+ "name": "Wori",
+ "pos_x": -155.34375,
+ "pos_y": 48.1875,
+ "pos_z": 7.78125,
+ "stations": "Erdos Landing,Kirk Vision,Kronecker Prospect"
+ },
+ {
+ "name": "Woria",
+ "pos_x": 127.96875,
+ "pos_y": -148.28125,
+ "pos_z": 31.65625,
+ "stations": "Roelofs Mines,Friend Terminal"
+ },
+ {
+ "name": "Worici",
+ "pos_x": -43.65625,
+ "pos_y": -72.8125,
+ "pos_z": 121.46875,
+ "stations": "Westerfeld Vista,Walter Mine"
+ },
+ {
+ "name": "Woriosolis",
+ "pos_x": -1.34375,
+ "pos_y": 106.84375,
+ "pos_z": 74.15625,
+ "stations": "Bugrov Freeport"
+ },
+ {
+ "name": "Worom Pef",
+ "pos_x": 62.875,
+ "pos_y": -29.34375,
+ "pos_z": 31.625,
+ "stations": "Stanley Settlement"
+ },
+ {
+ "name": "Woronii",
+ "pos_x": -32.5625,
+ "pos_y": -2.78125,
+ "pos_z": -130.25,
+ "stations": "Panshin Installation,Beaufoy Landing,Dietz Keep"
+ },
+ {
+ "name": "Woronir",
+ "pos_x": 136.625,
+ "pos_y": -149.28125,
+ "pos_z": 56.78125,
+ "stations": "Esclangon Vision,Dingle Silo,Whitcomb Station,Kaneda Orbital"
+ },
+ {
+ "name": "Worora",
+ "pos_x": 89.125,
+ "pos_y": 21.59375,
+ "pos_z": -55.21875,
+ "stations": "Lee Depot,Lonchakov Barracks,Asher Terminal"
+ },
+ {
+ "name": "Worotri",
+ "pos_x": 138.46875,
+ "pos_y": 51.6875,
+ "pos_z": -108.53125,
+ "stations": "Elcano Orbital,Oramus Orbital,Brin Base,McDonald City"
+ },
+ {
+ "name": "Wosraesi",
+ "pos_x": 58.1875,
+ "pos_y": 77.75,
+ "pos_z": -83.5625,
+ "stations": "Cormack Port,Lopez de Villalobos Hub,Treshchov Gateway,Levi-Montalcini Hub,Ford Port,Hoffman Terminal,Morrison Settlement,Heck Prospect"
+ },
+ {
+ "name": "Wosreti",
+ "pos_x": -20.625,
+ "pos_y": -98.03125,
+ "pos_z": 85.34375,
+ "stations": "Jackson Hub"
+ },
+ {
+ "name": "Wosyet",
+ "pos_x": 70.5,
+ "pos_y": -221,
+ "pos_z": 1.78125,
+ "stations": "Niemeyer Orbital,Molchanov Enterprise"
+ },
+ {
+ "name": "Wosyra Pao",
+ "pos_x": 11.53125,
+ "pos_y": 31.375,
+ "pos_z": -13.9375,
+ "stations": "Eyharts Station,Shargin Lab,Newton City,Lundwall Base"
+ },
+ {
+ "name": "Woyemsit",
+ "pos_x": -17.84375,
+ "pos_y": -107.71875,
+ "pos_z": 116.46875,
+ "stations": "Lewis Hub,Bolton Hub,Patry Hub,Andrews Vision,Watts Settlement"
+ },
+ {
+ "name": "Woyeru",
+ "pos_x": 77.5625,
+ "pos_y": -64.09375,
+ "pos_z": -160.15625,
+ "stations": "Artin Hub,Shosuke Bastion,Evangelisti Camp"
+ },
+ {
+ "name": "Woyet",
+ "pos_x": 62.46875,
+ "pos_y": -38.21875,
+ "pos_z": -74.78125,
+ "stations": "Burbank Horizons,Tolstoi's Inheritance"
+ },
+ {
+ "name": "Woyo Mina",
+ "pos_x": 113,
+ "pos_y": -90.46875,
+ "pos_z": 57.1875,
+ "stations": "Cannon Vision,MacCready Hub,Cartier Refinery,Cheranovsky Mines"
+ },
+ {
+ "name": "Wu Chelki",
+ "pos_x": -67.34375,
+ "pos_y": -182.875,
+ "pos_z": 23.3125,
+ "stations": "Parkinson City,Paola Station,De City,Kozlov Survey"
+ },
+ {
+ "name": "Wu Gondru",
+ "pos_x": -132.6875,
+ "pos_y": 23.15625,
+ "pos_z": -20.125,
+ "stations": "Celsius Enterprise,Precourt Beacon,Kovalevsky Mines"
+ },
+ {
+ "name": "Wu Guan",
+ "pos_x": 113.90625,
+ "pos_y": 96.03125,
+ "pos_z": 126.8125,
+ "stations": "Foden Dock,White Horizons,Leichhardt Vision,la Cosa Plant"
+ },
+ {
+ "name": "Wu Guinagi",
+ "pos_x": 60.28125,
+ "pos_y": -231.84375,
+ "pos_z": -53.40625,
+ "stations": "Ziegel Dock,Camm Enterprise,Bode Hub"
+ },
+ {
+ "name": "Wu Jinifex",
+ "pos_x": 103.9375,
+ "pos_y": -30,
+ "pos_z": 132.53125,
+ "stations": "Elson Point,Duque Relay"
+ },
+ {
+ "name": "Wu Jiu",
+ "pos_x": -146.21875,
+ "pos_y": 15.625,
+ "pos_z": 39.71875,
+ "stations": "Verrazzano Port,Cantor Vision,Kovalevskaya Barracks"
+ },
+ {
+ "name": "Wu Kich",
+ "pos_x": -119.8125,
+ "pos_y": -96.90625,
+ "pos_z": -37.15625,
+ "stations": "Bunch Enterprise,Tasman Vision,Nielsen Gateway,Darboux Colony"
+ },
+ {
+ "name": "Wu Kong",
+ "pos_x": -101.375,
+ "pos_y": -22.53125,
+ "pos_z": 56.6875,
+ "stations": "Ryman Dock,Schmidt Observatory,Asprin Station,Norman Horizons,Boulle's Progress"
+ },
+ {
+ "name": "Wu Ku",
+ "pos_x": 11.1875,
+ "pos_y": 64.375,
+ "pos_z": 114.71875,
+ "stations": "Vizcaino Refinery"
+ },
+ {
+ "name": "Wu Kui",
+ "pos_x": 15.0625,
+ "pos_y": -193.03125,
+ "pos_z": 119.90625,
+ "stations": "d'Arrest Colony,Schumacher Settlement,Kurtz Vision"
+ },
+ {
+ "name": "Wu Kuku",
+ "pos_x": 52.75,
+ "pos_y": -14.84375,
+ "pos_z": -18.53125,
+ "stations": "Al Saud Terminal,Buffett Port,Irvin Ring,Gerlache Barracks"
+ },
+ {
+ "name": "Wu Kwannya",
+ "pos_x": 54.5625,
+ "pos_y": -36.53125,
+ "pos_z": -98.09375,
+ "stations": "Shriver Vision"
+ },
+ {
+ "name": "Wu Te Ti",
+ "pos_x": 127.0625,
+ "pos_y": -95.40625,
+ "pos_z": 95.09375,
+ "stations": "von Biela City"
+ },
+ {
+ "name": "Wudjal",
+ "pos_x": -71.09375,
+ "pos_y": -36.0625,
+ "pos_z": -5.90625,
+ "stations": "Carstensz Port,Ozanne Enterprise,Murray Depot,Anderson Market,Vaez de Torres Dock,Euler Survey,Lenthall Dock,Fernandez Depot,Wallis Port,Scheerbart Hub,Kaleri Installation"
+ },
+ {
+ "name": "Wudjalie",
+ "pos_x": 36.3125,
+ "pos_y": -230.21875,
+ "pos_z": 45.40625,
+ "stations": "Bharadwaj Terminal,Kapteyn Station,Barnes Arsenal"
+ },
+ {
+ "name": "Wudjari",
+ "pos_x": 142.5625,
+ "pos_y": -210.4375,
+ "pos_z": -51.34375,
+ "stations": "Nakamura Works"
+ },
+ {
+ "name": "Wudji",
+ "pos_x": 73.125,
+ "pos_y": -222.03125,
+ "pos_z": -57.46875,
+ "stations": "la Cosa Landing,Pollas Holdings,Ahnert Colony"
+ },
+ {
+ "name": "Wuikito",
+ "pos_x": 9.875,
+ "pos_y": 3.03125,
+ "pos_z": 133.84375,
+ "stations": "Spielberg Orbital,Nixon Penal colony,Sagan Prospect"
+ },
+ {
+ "name": "Wulba",
+ "pos_x": -77.5,
+ "pos_y": 5.8125,
+ "pos_z": 124.40625,
+ "stations": "Rasch Port"
+ },
+ {
+ "name": "Wulgaedia",
+ "pos_x": -115.6875,
+ "pos_y": 55.3125,
+ "pos_z": -2.65625,
+ "stations": "Hale Station,Thuot Market,Egan Base,Lee Silo"
+ },
+ {
+ "name": "Wulganda",
+ "pos_x": -5.5625,
+ "pos_y": -0.90625,
+ "pos_z": 110.125,
+ "stations": "Cassidy Orbital,Hauck Orbital,Onizuka Mines,Swanson Terminal,Ogden Prospect"
+ },
+ {
+ "name": "Wulgarami",
+ "pos_x": 126.625,
+ "pos_y": 86.5,
+ "pos_z": 36.25,
+ "stations": "Ampere Survey,Wetherbee Dock,Langsdorff Installation"
+ },
+ {
+ "name": "Wulgu",
+ "pos_x": 150.9375,
+ "pos_y": -207.15625,
+ "pos_z": 48.84375,
+ "stations": "Camm Settlement"
+ },
+ {
+ "name": "Wuli",
+ "pos_x": -107.21875,
+ "pos_y": 71.96875,
+ "pos_z": 44.96875,
+ "stations": "Behnken Gateway,Rominger Laboratory,Vernadsky Laboratory"
+ },
+ {
+ "name": "Wulidjin",
+ "pos_x": -47.25,
+ "pos_y": -17.6875,
+ "pos_z": -128.21875,
+ "stations": "Nikolayev Ring,Currie Ring,Pelt Installation,Skvortsov City,Stein Works"
+ },
+ {
+ "name": "Wulis",
+ "pos_x": 13.4375,
+ "pos_y": 94.28125,
+ "pos_z": -101.03125,
+ "stations": "Hopper Enterprise,Aksyonov Ring,Thompson Relay"
+ },
+ {
+ "name": "Wulpa",
+ "pos_x": -126.09375,
+ "pos_y": -83.09375,
+ "pos_z": -85.5625,
+ "stations": "Williams Gateway"
+ },
+ {
+ "name": "Wulpulis",
+ "pos_x": -64.09375,
+ "pos_y": -15.71875,
+ "pos_z": 138.75,
+ "stations": "Ferguson Gateway,Dickson Prospect,Moore Enterprise,Hopkins Settlement"
+ },
+ {
+ "name": "Wulpurna",
+ "pos_x": 85.125,
+ "pos_y": 18.84375,
+ "pos_z": -52.21875,
+ "stations": "Patrick Ring,Lousma Enterprise,Favier Landing,Baker Survey,Volkov Arsenal"
+ },
+ {
+ "name": "Wuluang",
+ "pos_x": 126.46875,
+ "pos_y": -133.625,
+ "pos_z": -20.3125,
+ "stations": "Pannekoek Vision,Dreyer Beacon,Lopez Oasis"
+ },
+ {
+ "name": "Wulunges",
+ "pos_x": 171.9375,
+ "pos_y": -171.625,
+ "pos_z": -2.96875,
+ "stations": "Austin Mine"
+ },
+ {
+ "name": "Wulunghus",
+ "pos_x": 118.3125,
+ "pos_y": 34.90625,
+ "pos_z": -6.75,
+ "stations": "Thornycroft Plant,Dickson Landing,Hassanein Penal colony"
+ },
+ {
+ "name": "Wulungu",
+ "pos_x": 117.53125,
+ "pos_y": -0.75,
+ "pos_z": -119.09375,
+ "stations": "Lee Bastion,Moseley Port"
+ },
+ {
+ "name": "Wuluujjusi",
+ "pos_x": -86.4375,
+ "pos_y": -136,
+ "pos_z": 56.34375,
+ "stations": "Lavrador Vision,Stebler Bastion"
+ },
+ {
+ "name": "Wuluwait",
+ "pos_x": -5.4375,
+ "pos_y": -87.28125,
+ "pos_z": -55.03125,
+ "stations": "Sherrington Terminal,Crown Works"
+ },
+ {
+ "name": "Wulwul",
+ "pos_x": -123.75,
+ "pos_y": -46.65625,
+ "pos_z": 14.9375,
+ "stations": "Lawhead Ring,Kroehl Gateway"
+ },
+ {
+ "name": "Wulwula",
+ "pos_x": -39.03125,
+ "pos_y": -46.09375,
+ "pos_z": 102.28125,
+ "stations": "Fermi Dock,Lovelace Port,Levy Base,Asher's Folly,Foda Dock"
+ },
+ {
+ "name": "Wulwulan",
+ "pos_x": -89.625,
+ "pos_y": -76.09375,
+ "pos_z": -127.8125,
+ "stations": "Oramus Platform"
+ },
+ {
+ "name": "Wum",
+ "pos_x": 91.21875,
+ "pos_y": -64.5625,
+ "pos_z": 159.21875,
+ "stations": "Lichtenberg Dock,Raleigh Dock,Capek Arsenal,Delany Relay"
+ },
+ {
+ "name": "Wunab",
+ "pos_x": -84.625,
+ "pos_y": -41.1875,
+ "pos_z": -63.125,
+ "stations": "Lazutkin Vision"
+ },
+ {
+ "name": "Wunian",
+ "pos_x": -130.0625,
+ "pos_y": 18.375,
+ "pos_z": -32.9375,
+ "stations": "Potter Port,Burke Arsenal,Ford's Claim"
+ },
+ {
+ "name": "Wuniez",
+ "pos_x": 5.375,
+ "pos_y": -32.78125,
+ "pos_z": -84.5625,
+ "stations": "Lagrange Relay"
+ },
+ {
+ "name": "Wunii",
+ "pos_x": -6.25,
+ "pos_y": 31,
+ "pos_z": 166.21875,
+ "stations": "Adams Station,Wood Enterprise,Schmidt Enterprise,Narita's Progress"
+ },
+ {
+ "name": "Wuning",
+ "pos_x": 169.53125,
+ "pos_y": -20.4375,
+ "pos_z": -16.9375,
+ "stations": "Crampton Vision,Lee's Claim,Lee Relay"
+ },
+ {
+ "name": "Wuninka",
+ "pos_x": 120.84375,
+ "pos_y": -218.625,
+ "pos_z": 117.3125,
+ "stations": "Kobayashi Mine"
+ },
+ {
+ "name": "Wunitola",
+ "pos_x": -89.78125,
+ "pos_y": -65.4375,
+ "pos_z": -109.4375,
+ "stations": "Wisoff Enterprise,Scott Barracks,Lander Laboratory"
+ },
+ {
+ "name": "Wunjo",
+ "pos_x": -65,
+ "pos_y": 53.3125,
+ "pos_z": -22.59375,
+ "stations": "Baudin Hangar,Farmer Penitentiary"
+ },
+ {
+ "name": "Wuonai",
+ "pos_x": -36.46875,
+ "pos_y": 113.03125,
+ "pos_z": 72.125,
+ "stations": "Bardeen Station,Morris Landing"
+ },
+ {
+ "name": "Wuonanie",
+ "pos_x": 150.09375,
+ "pos_y": -157.09375,
+ "pos_z": 25.28125,
+ "stations": "Arai Station,Artzybasheff Station,Picacio Station,Adkins Dock,Schmidt Orbital,Youll Enterprise,Shosuke Depot,Curtis Point,Mayor Ring,Grant Station,Pilcher Penal colony,Asclepi's Progress"
+ },
+ {
+ "name": "Wuonjami",
+ "pos_x": -89.5,
+ "pos_y": -67.34375,
+ "pos_z": -36.625,
+ "stations": "Varley Beacon,Lu Refinery,Mendeleev Installation"
+ },
+ {
+ "name": "Wuonjulnju",
+ "pos_x": -140.0625,
+ "pos_y": -16.46875,
+ "pos_z": 2.125,
+ "stations": "Greenleaf Depot,Merle Survey"
+ },
+ {
+ "name": "Wuonjungu",
+ "pos_x": 56.75,
+ "pos_y": 22.59375,
+ "pos_z": -158.75,
+ "stations": "Campbell Escape"
+ },
+ {
+ "name": "Wuonjurubal",
+ "pos_x": 81.40625,
+ "pos_y": 42.03125,
+ "pos_z": -57.3125,
+ "stations": "Swainson Landing,Rennie Platform"
+ },
+ {
+ "name": "Wuonkwer",
+ "pos_x": 186.625,
+ "pos_y": -161.78125,
+ "pos_z": 35.9375,
+ "stations": "Mizuno Mines,Bernoulli Penal colony"
+ },
+ {
+ "name": "Wuonoru",
+ "pos_x": 92.65625,
+ "pos_y": -179.65625,
+ "pos_z": 113.84375,
+ "stations": "Alexander Camp"
+ },
+ {
+ "name": "Wurango",
+ "pos_x": 13.90625,
+ "pos_y": 136.90625,
+ "pos_z": -43.15625,
+ "stations": "Piaget Orbital,Markov Terminal,Amnuel Hub"
+ },
+ {
+ "name": "Wurr",
+ "pos_x": -33.78125,
+ "pos_y": -42.5625,
+ "pos_z": 69.28125,
+ "stations": "Patsayev Platform"
+ },
+ {
+ "name": "Wurra",
+ "pos_x": 146.125,
+ "pos_y": -198.0625,
+ "pos_z": -0.1875,
+ "stations": "Polikarpov Station"
+ },
+ {
+ "name": "Wurrucinga",
+ "pos_x": 48.59375,
+ "pos_y": -182.03125,
+ "pos_z": 31.65625,
+ "stations": "Bokeili Oasis,Hafner's Inheritance"
+ },
+ {
+ "name": "Wuru",
+ "pos_x": 31.03125,
+ "pos_y": -121.3125,
+ "pos_z": 22.8125,
+ "stations": "Urkovic Dock,Schuster Hub,McDaniel's Folly,Wendelin Terminal,Mattingly Forum,Paola Port,Leberecht Tempel Horizons,Brom City,Cogswell Relay"
+ },
+ {
+ "name": "Wuruacan",
+ "pos_x": 89.1875,
+ "pos_y": -119.40625,
+ "pos_z": 126.375,
+ "stations": "Paul Outpost,Parsons Depot"
+ },
+ {
+ "name": "Wuruku",
+ "pos_x": 95.15625,
+ "pos_y": -169,
+ "pos_z": 141.46875,
+ "stations": "Hussenot Platform,Wigura Settlement"
+ },
+ {
+ "name": "Wurun",
+ "pos_x": 221.78125,
+ "pos_y": -157.6875,
+ "pos_z": 73.84375,
+ "stations": "Okuni Terminal"
+ },
+ {
+ "name": "Wurunabijao",
+ "pos_x": 120.96875,
+ "pos_y": -132.03125,
+ "pos_z": 162.34375,
+ "stations": "Brunner Landing,Melotte Holdings"
+ },
+ {
+ "name": "Wurunsar",
+ "pos_x": 86.96875,
+ "pos_y": -120.5625,
+ "pos_z": -48.8125,
+ "stations": "Samos Terminal,Bova Barracks"
+ },
+ {
+ "name": "Wutha",
+ "pos_x": 139.84375,
+ "pos_y": -62.15625,
+ "pos_z": -9.875,
+ "stations": "Flammarion Vision,Tank Holdings,Butcher Point"
+ },
+ {
+ "name": "Wutha Gigae",
+ "pos_x": 88,
+ "pos_y": -26.6875,
+ "pos_z": 76.6875,
+ "stations": "Chiao Enterprise,Noon Penal colony"
+ },
+ {
+ "name": "Wuthawchu",
+ "pos_x": 107.875,
+ "pos_y": -22.21875,
+ "pos_z": -63.46875,
+ "stations": "Haignere Orbital,Tuan Station,Gardner Barracks,Grigson Camp"
+ },
+ {
+ "name": "Wuthielo Ku",
+ "pos_x": 68.3125,
+ "pos_y": -190.03125,
+ "pos_z": 12.375,
+ "stations": "Tarter Dock,Robert Dock,Mattingly Gateway"
+ },
+ {
+ "name": "Wuthnir",
+ "pos_x": -49.28125,
+ "pos_y": 122,
+ "pos_z": -18.09375,
+ "stations": "Haldeman II Horizons"
+ },
+ {
+ "name": "Wuthudiya",
+ "pos_x": -62.78125,
+ "pos_y": -122.59375,
+ "pos_z": 77.34375,
+ "stations": "Tilman City,Theodorsen Vision,Siegel Reach"
+ },
+ {
+ "name": "Wuy jugua",
+ "pos_x": -24.4375,
+ "pos_y": -125.4375,
+ "pos_z": -30.59375,
+ "stations": "Buckland Station"
+ },
+ {
+ "name": "Wuy jugun",
+ "pos_x": 116,
+ "pos_y": -41.875,
+ "pos_z": -12.09375,
+ "stations": "Shen Station"
+ },
+ {
+ "name": "Wuy jugurra",
+ "pos_x": -82.5,
+ "pos_y": -142.03125,
+ "pos_z": 15.78125,
+ "stations": "Dalton Landing,Broglie Colony"
+ },
+ {
+ "name": "WW Piscis Austrini",
+ "pos_x": -7.9375,
+ "pos_y": -68.46875,
+ "pos_z": 34.40625,
+ "stations": "Ivins Vision,Garan Ring,Sevastyanov Terminal,Hunt Bastion,Serling's Progress"
+ },
+ {
+ "name": "Wyrd",
+ "pos_x": -11.625,
+ "pos_y": 31.53125,
+ "pos_z": -3.9375,
+ "stations": "Gareth Edwards Park,Black Hide,Vonarburg Co-operative,Bokeili Station,Griggs Horizons"
+ },
+ {
+ "name": "Xakria",
+ "pos_x": 138.40625,
+ "pos_y": -198.28125,
+ "pos_z": 72.53125,
+ "stations": "Canty Orbital,Corben Port,Kushida Hub,Messerschmitt Orbital"
+ },
+ {
+ "name": "Xamen Ek",
+ "pos_x": -98.28125,
+ "pos_y": 37.84375,
+ "pos_z": 85.28125,
+ "stations": "Brunel Dock,Faraday Enterprise,Sharma Port,Vinci Port,Levi-Strauss Orbital,Burbank Station,Linenger Gateway,Fulton Hub,Parise Terminal,Bretnor Terminal,Kippax Relay"
+ },
+ {
+ "name": "Xamentii",
+ "pos_x": -52.15625,
+ "pos_y": 44.59375,
+ "pos_z": 90.34375,
+ "stations": "Busch Platform,Jordan Installation,Nagata Point"
+ },
+ {
+ "name": "Xang Tzu",
+ "pos_x": -12.96875,
+ "pos_y": -27.03125,
+ "pos_z": -88.3125,
+ "stations": "Lister Station"
+ },
+ {
+ "name": "Xangwe",
+ "pos_x": -107.34375,
+ "pos_y": 20.5,
+ "pos_z": 1,
+ "stations": "Euclid Hub,Laplace Enterprise,Cartwright Market,Makarov Station,Dirac Station,Cardano Dock,Vetulani Hub,Jones Holdings"
+ },
+ {
+ "name": "Xava",
+ "pos_x": 7.78125,
+ "pos_y": -118.96875,
+ "pos_z": 128.90625,
+ "stations": "Bentham Installation,Wirtanen Camp"
+ },
+ {
+ "name": "Xavalenti",
+ "pos_x": -67.40625,
+ "pos_y": 98.78125,
+ "pos_z": 102.9375,
+ "stations": "Hope Beacon,Joliot-Curie's Claim"
+ },
+ {
+ "name": "Xavane",
+ "pos_x": -132.15625,
+ "pos_y": 44.78125,
+ "pos_z": 70.125,
+ "stations": "Merchiston Horizons,Carpenter Survey"
+ },
+ {
+ "name": "Xbal",
+ "pos_x": -40.65625,
+ "pos_y": 60.6875,
+ "pos_z": -123.46875,
+ "stations": "Edwards Horizons,Williams Vision,Shaw Terminal,Merle Lab"
+ },
+ {
+ "name": "Xbalamana",
+ "pos_x": 47.3125,
+ "pos_y": -217.53125,
+ "pos_z": 39.6875,
+ "stations": "Winthrop Gateway,Gehry Port,Littrow's Progress,Baird Settlement,Lindblad's Folly"
+ },
+ {
+ "name": "Xbaquitae",
+ "pos_x": 79.28125,
+ "pos_y": 18.1875,
+ "pos_z": -156.8125,
+ "stations": "Snodgrass Orbital,Hahn Colony"
+ },
+ {
+ "name": "Xelabara",
+ "pos_x": -93.40625,
+ "pos_y": 66.0625,
+ "pos_z": -44.96875,
+ "stations": "Navigator Market,Morris Prospect,North Prospect,Bounds' Claim"
+ },
+ {
+ "name": "Xelala",
+ "pos_x": -76.5625,
+ "pos_y": 160.1875,
+ "pos_z": -37.25,
+ "stations": "Alvarez de Pineda Landing"
+ },
+ {
+ "name": "Xelardi",
+ "pos_x": 72.875,
+ "pos_y": -20.59375,
+ "pos_z": 84.53125,
+ "stations": "Huggins Keep,Steakley Station,Kube-McDowell Settlement"
+ },
+ {
+ "name": "Xelas",
+ "pos_x": 20.5,
+ "pos_y": -198.375,
+ "pos_z": 133.65625,
+ "stations": "Duque Outpost,Webb Relay"
+ },
+ {
+ "name": "Xerebaye",
+ "pos_x": 104.65625,
+ "pos_y": 91,
+ "pos_z": 9.25,
+ "stations": "King Landing,Neumann Settlement,Vaez de Torres Landing,Morgan Depot"
+ },
+ {
+ "name": "Xerente",
+ "pos_x": 96.75,
+ "pos_y": -125.5,
+ "pos_z": 83.8125,
+ "stations": "Krenkel Horizons,Berkey Dock,Miyasaka Barracks"
+ },
+ {
+ "name": "Xeret",
+ "pos_x": 72.6875,
+ "pos_y": -180.25,
+ "pos_z": 164.5625,
+ "stations": "Smith Stop,Toll Barracks"
+ },
+ {
+ "name": "Xereta",
+ "pos_x": 61.46875,
+ "pos_y": -98.125,
+ "pos_z": -26.5625,
+ "stations": "Hoerner Terminal"
+ },
+ {
+ "name": "Xeretes",
+ "pos_x": -73.625,
+ "pos_y": -7.75,
+ "pos_z": 157.15625,
+ "stations": "Smith Stop,Cugnot Retreat"
+ },
+ {
+ "name": "Xevioso",
+ "pos_x": 0.84375,
+ "pos_y": 60.0625,
+ "pos_z": 24.15625,
+ "stations": "Avogadro Port,Ciferri Gateway,Armstrong Gateway,Clement Mines,Veach Hub"
+ },
+ {
+ "name": "Xhosag",
+ "pos_x": 46.625,
+ "pos_y": -100.75,
+ "pos_z": 68.125,
+ "stations": "Parsons Refinery,Bickel Vision"
+ },
+ {
+ "name": "Xhosattu",
+ "pos_x": 109.25,
+ "pos_y": 2.4375,
+ "pos_z": 63.59375,
+ "stations": "Mille Holdings,Alexandria Ring,Obruchev Camp,Lambert Holdings,Thierree Station,Fox Vision"
+ },
+ {
+ "name": "Xi Hara",
+ "pos_x": 79.1875,
+ "pos_y": -137.6875,
+ "pos_z": 50.71875,
+ "stations": "Hadid City,Roskam Landing,Ron Hubbard Holdings"
+ },
+ {
+ "name": "Xi Hesates",
+ "pos_x": -60.21875,
+ "pos_y": 27.28125,
+ "pos_z": -89.8125,
+ "stations": "Euthymenes Ring,Lopez de Villalobos Works,Crown Arsenal,Jones Keep"
+ },
+ {
+ "name": "Xi Hsinahau",
+ "pos_x": 69.40625,
+ "pos_y": -238.0625,
+ "pos_z": -1.53125,
+ "stations": "al-Kashi Survey,Mattingly Orbital"
+ },
+ {
+ "name": "Xi Hungan",
+ "pos_x": -75.46875,
+ "pos_y": 9.375,
+ "pos_z": -65.21875,
+ "stations": "Kekule Dock,Shaw Plant,Rand's Progress"
+ },
+ {
+ "name": "Xi Hydrae",
+ "pos_x": 110.625,
+ "pos_y": 60.9375,
+ "pos_z": 29.09375,
+ "stations": "Feustel Port,Chomsky Dock,Citi Holdings,Henry Orbital,Wingqvist Hub,Wetherbee Station,Griffith Survey,Arrhenius City,Abe Vision,Lupoff Hub"
+ },
+ {
+ "name": "Xi Ophiuchi",
+ "pos_x": -3.875,
+ "pos_y": 8.15625,
+ "pos_z": 55.875,
+ "stations": "Parazynski Station,Shargin Orbital,Khan Dock,Gell-Mann Orbital,Vian Ring,Wilmore Orbital,Varthema Reach,Fincke Station,Butler Holdings"
+ },
+ {
+ "name": "Xi Phoenicis",
+ "pos_x": 87.09375,
+ "pos_y": -190.96875,
+ "pos_z": 61.09375,
+ "stations": "Banno Penal colony,Edwards' Inheritance,Nylund Enterprise"
+ },
+ {
+ "name": "Xi Saon",
+ "pos_x": -72.90625,
+ "pos_y": 18.59375,
+ "pos_z": -114.8125,
+ "stations": "Gillekens Bastion,Cavendish Enterprise,Rutherford Enterprise,Atkov Port,Elion Relay"
+ },
+ {
+ "name": "Xi Shan",
+ "pos_x": 155,
+ "pos_y": 84.625,
+ "pos_z": -50.28125,
+ "stations": "Mieville Hub,Green Hub,Stafford Depot"
+ },
+ {
+ "name": "Xi Ursae Majoris",
+ "pos_x": 2.65625,
+ "pos_y": 27.09375,
+ "pos_z": -9.9375,
+ "stations": "Patrick Terminal,Durrance Dock"
+ },
+ {
+ "name": "Xi Wang Mu",
+ "pos_x": -36.46875,
+ "pos_y": -122.40625,
+ "pos_z": -44.4375,
+ "stations": "Goddard Dock,Khayyam Dock,Marshall Enterprise,Polansky Enterprise,Hopkins Terminal,Kovalevsky Dock,Avicenna Orbital,Johnson Enterprise,Shargin Enterprise,Offutt Barracks,Kapp Refinery"
+ },
+ {
+ "name": "Xi Wangar",
+ "pos_x": 9.21875,
+ "pos_y": -144.46875,
+ "pos_z": -0.53125,
+ "stations": "Thiele Camp"
+ },
+ {
+ "name": "Xi Wangda",
+ "pos_x": -21.8125,
+ "pos_y": -167,
+ "pos_z": -19.71875,
+ "stations": "Thollon Enterprise,Pippin Enterprise,Cartier City,Corben Vision,Tully's Inheritance"
+ },
+ {
+ "name": "Xi Wangkala",
+ "pos_x": -135.65625,
+ "pos_y": 35.3125,
+ "pos_z": -29.84375,
+ "stations": "Hill Legacy"
+ },
+ {
+ "name": "Xi Wanja",
+ "pos_x": -125.40625,
+ "pos_y": 32.59375,
+ "pos_z": 114.625,
+ "stations": "Chretien Settlement,Lenthall Survey"
+ },
+ {
+ "name": "Xi-2 Capricorni",
+ "pos_x": -42.3125,
+ "pos_y": -36.96875,
+ "pos_z": 70.75,
+ "stations": "Baird Gateway,Bouch Terminal,Lewitt Hub,Heinlein Survey,Worden City"
+ },
+ {
+ "name": "Xi-2 Lupi",
+ "pos_x": 59.59375,
+ "pos_y": 48.0625,
+ "pos_z": 180.40625,
+ "stations": "Williams Lab,Pellegrino Hub,Moorcock Survey,Soldier's Respite"
+ },
+ {
+ "name": "Xiancheim",
+ "pos_x": 137.5625,
+ "pos_y": -96.9375,
+ "pos_z": 28.4375,
+ "stations": "Hogg Survey,Hamuy Port,Resnick Point"
+ },
+ {
+ "name": "Xians",
+ "pos_x": -100.25,
+ "pos_y": -17.09375,
+ "pos_z": -28.09375,
+ "stations": "Lerman Dock,Garnier Vision,Isherwood Base"
+ },
+ {
+ "name": "Xib Cail",
+ "pos_x": 67.53125,
+ "pos_y": -19.71875,
+ "pos_z": 22.375,
+ "stations": "Lyell Laboratory,Coats Camp,Porsche Relay"
+ },
+ {
+ "name": "Xib Cakurbi",
+ "pos_x": 183.3125,
+ "pos_y": -166.75,
+ "pos_z": 26.09375,
+ "stations": "Balog Works,Michael Point"
+ },
+ {
+ "name": "Xibalba",
+ "pos_x": 87.9375,
+ "pos_y": -125.5,
+ "pos_z": 62.4375,
+ "stations": "Erikson Terminal,Fraunhofer Platform,Thollon Bastion"
+ },
+ {
+ "name": "Xibe",
+ "pos_x": -112.5,
+ "pos_y": 33.8125,
+ "pos_z": -60.03125,
+ "stations": "Wood City,Lagrange City,Willis Orbital,McDaniel Installation,Thurston Orbital,Russ Vision,Salgari Laboratory,Banks Survey,Kennan's Progress"
+ },
+ {
+ "name": "Xihe",
+ "pos_x": -19.8125,
+ "pos_y": -35.375,
+ "pos_z": 34,
+ "stations": "Shepherd Silo,Zhen Dock,Langford Survey"
+ },
+ {
+ "name": "Xinca",
+ "pos_x": 123.90625,
+ "pos_y": -6.40625,
+ "pos_z": -48.9375,
+ "stations": "Stross Hub,Finch Market,Fremont Landing,Snodgrass Enterprise,Niven Dock,Smith Orbital,Coelho Hub,Smith City,Beebe Vista,Alpers Penal colony"
+ },
+ {
+ "name": "Xingait",
+ "pos_x": -62.75,
+ "pos_y": -33.125,
+ "pos_z": 90.96875,
+ "stations": "Staden's Claim,Baraniecki Asylum,Matteucci Enterprise"
+ },
+ {
+ "name": "Xingang Mu",
+ "pos_x": -19.96875,
+ "pos_y": -152.1875,
+ "pos_z": 109.03125,
+ "stations": "Backlund Survey,Andrews Refinery"
+ },
+ {
+ "name": "Xingara",
+ "pos_x": -48.71875,
+ "pos_y": -86.5625,
+ "pos_z": 19.71875,
+ "stations": "Mondeh Terminal,Einstein Hub,Scully-Power Settlement"
+ },
+ {
+ "name": "Xingaroans",
+ "pos_x": 97.4375,
+ "pos_y": -134.90625,
+ "pos_z": 82.84375,
+ "stations": "Bisnovatyi-Kogan Mines"
+ },
+ {
+ "name": "Xingga",
+ "pos_x": 67.5,
+ "pos_y": 8.625,
+ "pos_z": -22.15625,
+ "stations": "Olsen Station,Pythagoras Port,Steele Base"
+ },
+ {
+ "name": "Xion",
+ "pos_x": 91.1875,
+ "pos_y": -72.53125,
+ "pos_z": 119.5,
+ "stations": "Muramatsu Vision,Sabine Gateway,Arago City,Fast Reach"
+ },
+ {
+ "name": "Xionaz",
+ "pos_x": 0.96875,
+ "pos_y": 29.96875,
+ "pos_z": -153.59375,
+ "stations": "Hurston Settlement,Carr Beacon,Timofeyevich Beacon"
+ },
+ {
+ "name": "Xionean",
+ "pos_x": -13.6875,
+ "pos_y": -136.0625,
+ "pos_z": 108.3125,
+ "stations": "Unsold Gateway,Carsono Terminal"
+ },
+ {
+ "name": "Xiongandi",
+ "pos_x": 33.90625,
+ "pos_y": 13.53125,
+ "pos_z": 164.25,
+ "stations": "Wnuk-Lipinski Freeport,Kirtley Prospect"
+ },
+ {
+ "name": "Xiongga",
+ "pos_x": 66.6875,
+ "pos_y": -159.5,
+ "pos_z": 57.5,
+ "stations": "Kandrup Survey"
+ },
+ {
+ "name": "Xiri",
+ "pos_x": 32.125,
+ "pos_y": -129.28125,
+ "pos_z": 101.875,
+ "stations": "Anderson Installation"
+ },
+ {
+ "name": "Xiripa",
+ "pos_x": 30.5625,
+ "pos_y": -1.09375,
+ "pos_z": -137.96875,
+ "stations": "Kanwar Gateway,Vasilyev Hub,Lyell Port,Atwater Port,Euclid Camp"
+ },
+ {
+ "name": "Xiriwal",
+ "pos_x": 99.84375,
+ "pos_y": 76.375,
+ "pos_z": -3.8125,
+ "stations": "Horch Dock,Mouhot Hub"
+ },
+ {
+ "name": "Xiriyanoar",
+ "pos_x": -126.40625,
+ "pos_y": 91.65625,
+ "pos_z": 5.9375,
+ "stations": "Soto Hub"
+ },
+ {
+ "name": "Xmucainakun",
+ "pos_x": 118.3125,
+ "pos_y": 17.78125,
+ "pos_z": -3.9375,
+ "stations": "Laval Mines,Sinclair Port,Anthony Prospect"
+ },
+ {
+ "name": "Xmucanoe",
+ "pos_x": 105.3125,
+ "pos_y": 71.3125,
+ "pos_z": 110.09375,
+ "stations": "Solovyov Camp,Eisele Outpost,Wingqvist Depot"
+ },
+ {
+ "name": "Xolotl",
+ "pos_x": -21.53125,
+ "pos_y": 43.5,
+ "pos_z": -41.65625,
+ "stations": "Ore Refinery,Warner Landing,Eskridge Beacon,Grimwood Colony"
+ },
+ {
+ "name": "Xpiyacoc",
+ "pos_x": 103.8125,
+ "pos_y": -32,
+ "pos_z": 129.84375,
+ "stations": "Wolf Relay"
+ },
+ {
+ "name": "Xuan",
+ "pos_x": -76.6875,
+ "pos_y": -74.625,
+ "pos_z": 115.5,
+ "stations": "McCaffrey's Folly,Wells Point"
+ },
+ {
+ "name": "Xuan Chelg",
+ "pos_x": -119.5625,
+ "pos_y": -55.53125,
+ "pos_z": 70.53125,
+ "stations": "Bessemer Landing,Tepper Refinery,Walker Enterprise,Eudoxus' Inheritance"
+ },
+ {
+ "name": "Xuan Wena",
+ "pos_x": -51.25,
+ "pos_y": 78.21875,
+ "pos_z": -58.1875,
+ "stations": "Paez Freeport,Henslow Colony"
+ },
+ {
+ "name": "Xuanars",
+ "pos_x": -107.9375,
+ "pos_y": -132.9375,
+ "pos_z": 12.4375,
+ "stations": "Bresnik Mines,Kozlov's Claim"
+ },
+ {
+ "name": "Xuanduna",
+ "pos_x": 111.03125,
+ "pos_y": -55.59375,
+ "pos_z": 27.5625,
+ "stations": "Furukawa Station,De Lay Terminal,Gehry Dock,Check Ring,Cousin Point,Prandtl Prospect,Sugano Orbital"
+ },
+ {
+ "name": "Xuane",
+ "pos_x": 93.875,
+ "pos_y": -9.25,
+ "pos_z": -32.53125,
+ "stations": "Goeppert-Mayer Station,Mondeh Station,Ramsbottom Hub,Pontes Hub"
+ },
+ {
+ "name": "Xuanebuth",
+ "pos_x": 114.59375,
+ "pos_y": -112.0625,
+ "pos_z": -21.46875,
+ "stations": "Adelman Palace,Shoujing Oasis"
+ },
+ {
+ "name": "Xuanec",
+ "pos_x": -60.1875,
+ "pos_y": -43.4375,
+ "pos_z": 124.9375,
+ "stations": "Ibold Port,Lander Gateway,Vespucci Orbital,Narita's Progress"
+ },
+ {
+ "name": "Xuang",
+ "pos_x": -120.34375,
+ "pos_y": 97.9375,
+ "pos_z": 92.46875,
+ "stations": "Great Platform"
+ },
+ {
+ "name": "Xuang Wa",
+ "pos_x": 119.65625,
+ "pos_y": -104.71875,
+ "pos_z": -37.125,
+ "stations": "Smirnova Stop"
+ },
+ {
+ "name": "Xuangeriva",
+ "pos_x": 212.40625,
+ "pos_y": -136.625,
+ "pos_z": 73.8125,
+ "stations": "Bond Prospect,Lopez de Haro Depot"
+ },
+ {
+ "name": "Xuangkomi",
+ "pos_x": -117.4375,
+ "pos_y": -32.03125,
+ "pos_z": 34.9375,
+ "stations": "Resnick Mines"
+ },
+ {
+ "name": "Xuangorona",
+ "pos_x": -163.21875,
+ "pos_y": -40.96875,
+ "pos_z": 26.125,
+ "stations": "Agnesi Port,Walker Terminal"
+ },
+ {
+ "name": "Xuangu",
+ "pos_x": 122.1875,
+ "pos_y": -33.40625,
+ "pos_z": 67.375,
+ "stations": "Schroter Terminal,Moffitt Town,Spielberg Keep,Merritt Vision"
+ },
+ {
+ "name": "Xuanook",
+ "pos_x": 8.9375,
+ "pos_y": -77.9375,
+ "pos_z": -95.96875,
+ "stations": "Kondratyev Landing,Ramaswamy Dock,Zamka Works,Ballard Refinery"
+ },
+ {
+ "name": "Xucumandji",
+ "pos_x": 68.40625,
+ "pos_y": 144.40625,
+ "pos_z": 91.3125,
+ "stations": "Yu Legacy,Ballard Landing"
+ },
+ {
+ "name": "Xucuri",
+ "pos_x": 60.90625,
+ "pos_y": 56.03125,
+ "pos_z": 53.15625,
+ "stations": "Al-Farabi Hangar,Halsell Colony,Kozeyev Penal colony"
+ },
+ {
+ "name": "Xue Davokje",
+ "pos_x": 88.25,
+ "pos_y": -102.15625,
+ "pos_z": -70.78125,
+ "stations": "Adelman's Folly,Seamans Hub,Ludwig Struve Gateway,Tsiolkovsky Orbital"
+ },
+ {
+ "name": "Xue Deng",
+ "pos_x": 84,
+ "pos_y": -13.5625,
+ "pos_z": 26.3125,
+ "stations": "Babcock Landing,Arkwright Hub"
+ },
+ {
+ "name": "Xue Zhan",
+ "pos_x": -65.6875,
+ "pos_y": -27,
+ "pos_z": 141.125,
+ "stations": "Hurley Installation,Ockels Depot"
+ },
+ {
+ "name": "XZ Leonis Minoris",
+ "pos_x": 25.4375,
+ "pos_y": 106.3125,
+ "pos_z": -41.25,
+ "stations": "Webb Survey,Abel Platform,Doctorow Beacon"
+ },
+ {
+ "name": "Y Velorum",
+ "pos_x": 69.03125,
+ "pos_y": 4.4375,
+ "pos_z": 16.46875,
+ "stations": "Irvin Hub,Turzillo Enterprise,Vaugh Orbital"
+ },
+ {
+ "name": "Ya'Noma",
+ "pos_x": 130.71875,
+ "pos_y": 27.9375,
+ "pos_z": 66.875,
+ "stations": "Zhen Settlement,Good Colony"
+ },
+ {
+ "name": "Ya'Nomamo",
+ "pos_x": 60.96875,
+ "pos_y": -163.46875,
+ "pos_z": -31.5,
+ "stations": "Ptack Landing,Loewy Port,Bowell Colony"
+ },
+ {
+ "name": "Yab Camalo",
+ "pos_x": 81.84375,
+ "pos_y": -180.125,
+ "pos_z": -14.6875,
+ "stations": "Miller Ring,Kidd City,Muller Station,von Zach Hub,Weill Hub,Montanari Survey,Grigson Point,Celebi Vision"
+ },
+ {
+ "name": "Yab Cameni",
+ "pos_x": 99.4375,
+ "pos_y": -73.53125,
+ "pos_z": 12.6875,
+ "stations": "Steiner Settlement,South Orbital,McQuarrie Dock,Brust's Folly,Olahus Holdings"
+ },
+ {
+ "name": "Yab Yum",
+ "pos_x": 50.71875,
+ "pos_y": -156.90625,
+ "pos_z": -12.375,
+ "stations": "Abetti Vision,Carpenter City,Lagadha Terminal,Clark Ring,Jones Station,Schmidt Gateway,Rochon Settlement,Malcolm Relay,Ortiz Moreno Depot,Pellegrino Landing,Nelder Enterprise,Danjon Holdings,Sitter Gateway,Rosseland Terminal,Phillips Vision"
+ },
+ {
+ "name": "Yadhariu",
+ "pos_x": -96.3125,
+ "pos_y": 34.0625,
+ "pos_z": 68.90625,
+ "stations": "Berezovoy Hub"
+ },
+ {
+ "name": "Yaghan",
+ "pos_x": 24.84375,
+ "pos_y": 99.0625,
+ "pos_z": 55.5625,
+ "stations": "Berezin Station,Abe Terminal,Moskowitz Enterprise,Lichtenberg Gateway"
+ },
+ {
+ "name": "Yaguvit",
+ "pos_x": 37.15625,
+ "pos_y": -159.40625,
+ "pos_z": 116.125,
+ "stations": "Mikoyan Relay"
+ },
+ {
+ "name": "Yakabugai",
+ "pos_x": -43.84375,
+ "pos_y": -7.625,
+ "pos_z": 64.78125,
+ "stations": "Levi-Montalcini City,Serebrov Station,Aristotle Gateway"
+ },
+ {
+ "name": "Yakaeriate",
+ "pos_x": 80.28125,
+ "pos_y": -21.5625,
+ "pos_z": 100.84375,
+ "stations": "Gresley Ring,Forward Observatory"
+ },
+ {
+ "name": "Yakaldukpe",
+ "pos_x": -1.78125,
+ "pos_y": 54.75,
+ "pos_z": 156.375,
+ "stations": "Anderson Platform"
+ },
+ {
+ "name": "Yakama",
+ "pos_x": 23.03125,
+ "pos_y": -50.375,
+ "pos_z": 107.53125,
+ "stations": "Ricardo Enterprise"
+ },
+ {
+ "name": "Yakamu",
+ "pos_x": -141.25,
+ "pos_y": -4.59375,
+ "pos_z": 73.9375,
+ "stations": "Lyell Port,Kanwar Station,Lubin Station,Jones Bastion"
+ },
+ {
+ "name": "Yakamutii",
+ "pos_x": 141.375,
+ "pos_y": -183.65625,
+ "pos_z": 122.46875,
+ "stations": "Hubble Terminal,Derekas Survey,Gaensler Base"
+ },
+ {
+ "name": "Yakat",
+ "pos_x": -108.28125,
+ "pos_y": -27.375,
+ "pos_z": 70.8125,
+ "stations": "Gardner Landing,Gaultier de Varennes Holdings"
+ },
+ {
+ "name": "Yakawana",
+ "pos_x": -103.09375,
+ "pos_y": 35.46875,
+ "pos_z": -31.28125,
+ "stations": "Yourkevitch Platform,Thurston Dock,Boole Estate"
+ },
+ {
+ "name": "Yaksa",
+ "pos_x": 119.21875,
+ "pos_y": -70.90625,
+ "pos_z": -12.0625,
+ "stations": "Beg Outpost"
+ },
+ {
+ "name": "Yaksara",
+ "pos_x": -30.46875,
+ "pos_y": 7.03125,
+ "pos_z": 107.21875,
+ "stations": "Bell Terminal,Novitski Orbital,Cheli Landing"
+ },
+ {
+ "name": "Yakshapoos",
+ "pos_x": -106.78125,
+ "pos_y": -48.125,
+ "pos_z": 108.6875,
+ "stations": "Gardner Mines"
+ },
+ {
+ "name": "Yakshmi",
+ "pos_x": 159.34375,
+ "pos_y": 50.0625,
+ "pos_z": -57.09375,
+ "stations": "Wait Colburn Hub,Scalzi Colony,Stjepan Seljan Settlement,Lichtenberg Mines"
+ },
+ {
+ "name": "Yalua",
+ "pos_x": 111.21875,
+ "pos_y": -142.90625,
+ "pos_z": 162.40625,
+ "stations": "Gaensler Outpost"
+ },
+ {
+ "name": "Yalung Ti",
+ "pos_x": -6.46875,
+ "pos_y": -165.4375,
+ "pos_z": 40.78125,
+ "stations": "Kawasato Colony,Impey Survey,Macan's Progress"
+ },
+ {
+ "name": "Yalungurawa",
+ "pos_x": -79.53125,
+ "pos_y": -82.25,
+ "pos_z": -81.15625,
+ "stations": "Sadi Carnot Port,Finney Beacon"
+ },
+ {
+ "name": "Yalura",
+ "pos_x": 12.40625,
+ "pos_y": -63.53125,
+ "pos_z": 78.75,
+ "stations": "Zoline Plant,Johnson Point,Miller Settlement"
+ },
+ {
+ "name": "Yaluwacha",
+ "pos_x": 64.125,
+ "pos_y": 9.84375,
+ "pos_z": -64.21875,
+ "stations": "Feynman Horizons,Walters Relay"
+ },
+ {
+ "name": "Yam",
+ "pos_x": 51,
+ "pos_y": -27.09375,
+ "pos_z": -50.75,
+ "stations": "Bethe Gateway,Kanwar City,Chargaff Ring,Duke Ring,Crown Orbital,Henricks Orbital,Brunton Hub,Rose Depot,Cernan Terminal,Ehrenfried Kegel Legacy,Vogel's Pride,Still Legacy,Butcher Settlement"
+ },
+ {
+ "name": "Yama",
+ "pos_x": 98.1875,
+ "pos_y": 26.46875,
+ "pos_z": 85,
+ "stations": "Pinto Co-operative,Akers Hub,Gerrold Depot"
+ },
+ {
+ "name": "Yamahun",
+ "pos_x": -11.15625,
+ "pos_y": -43.625,
+ "pos_z": -70.25,
+ "stations": "Hurston Platform"
+ },
+ {
+ "name": "Yamandara",
+ "pos_x": 80.3125,
+ "pos_y": -0.75,
+ "pos_z": 115.4375,
+ "stations": "Eilenberg Refinery,Herbert Beacon"
+ },
+ {
+ "name": "Yamari",
+ "pos_x": 82.15625,
+ "pos_y": -258.75,
+ "pos_z": -1.4375,
+ "stations": "Struzan Stop"
+ },
+ {
+ "name": "Yamasan",
+ "pos_x": 75.1875,
+ "pos_y": -10.3125,
+ "pos_z": -62.78125,
+ "stations": "Stairs Barracks,Aldrin Landing,Coke Refinery"
+ },
+ {
+ "name": "Yamatji",
+ "pos_x": 80.5625,
+ "pos_y": -11.34375,
+ "pos_z": -98.90625,
+ "stations": "Warner Gateway,Legendre Port,Gunn Dock,la Cosa Terminal,Chios Enterprise,Kiernan Hub,Webb Terminal,Peary Depot"
+ },
+ {
+ "name": "Yamatri",
+ "pos_x": 69.59375,
+ "pos_y": -177.5625,
+ "pos_z": -30.0625,
+ "stations": "Slonczewski Survey,Jones Colony,Ayers Station"
+ },
+ {
+ "name": "Yamatzicni",
+ "pos_x": 40.5,
+ "pos_y": -115.25,
+ "pos_z": 39.4375,
+ "stations": "Hirayama Platform"
+ },
+ {
+ "name": "Yami",
+ "pos_x": -111.03125,
+ "pos_y": 103.65625,
+ "pos_z": 32.03125,
+ "stations": "Aksyonov Platform"
+ },
+ {
+ "name": "Yaming",
+ "pos_x": -40.6875,
+ "pos_y": 102.0625,
+ "pos_z": -79.53125,
+ "stations": "Makeev Orbital,Eschbach Dock,Garnier Terminal,Mitchell Market,Cummings Terminal,Serre Bastion"
+ },
+ {
+ "name": "Yamuluntii",
+ "pos_x": 129.03125,
+ "pos_y": -53.3125,
+ "pos_z": -33.53125,
+ "stations": "Bao Base,Yano Reach"
+ },
+ {
+ "name": "Yamunab Yu",
+ "pos_x": -82.59375,
+ "pos_y": -0.21875,
+ "pos_z": 68.6875,
+ "stations": "Aleksandrov Terminal,Copernicus Orbital,Stirling Lab"
+ },
+ {
+ "name": "Yamur",
+ "pos_x": -14.625,
+ "pos_y": -18.25,
+ "pos_z": -179.25,
+ "stations": "Haber Installation,Younghusband Port,Vespucci Enterprise"
+ },
+ {
+ "name": "Yan Crua",
+ "pos_x": 14.40625,
+ "pos_y": 37.625,
+ "pos_z": 160.1875,
+ "stations": "Strzelecki Settlement,Plucker Settlement"
+ },
+ {
+ "name": "Yan Musu",
+ "pos_x": 107.21875,
+ "pos_y": -71.03125,
+ "pos_z": 122.8125,
+ "stations": "Norman Barracks,Sutter Dock,MacVicar Base,Cuffey Vision,Dunlop Terminal"
+ },
+ {
+ "name": "Yan Yi",
+ "pos_x": -145.5,
+ "pos_y": 24.21875,
+ "pos_z": -19.25,
+ "stations": "Jolliet Port,Wingrove Landing,Burnham Prospect"
+ },
+ {
+ "name": "Yan Yun Oya",
+ "pos_x": 29.1875,
+ "pos_y": 66.96875,
+ "pos_z": 62.15625,
+ "stations": "Henslow Works"
+ },
+ {
+ "name": "Yan Zangata",
+ "pos_x": 157.3125,
+ "pos_y": -77.59375,
+ "pos_z": -43.125,
+ "stations": "Naubakht Station,Hogg Station"
+ },
+ {
+ "name": "Yanebdjeri",
+ "pos_x": 159.875,
+ "pos_y": -220.3125,
+ "pos_z": 38.6875,
+ "stations": "Payne-Scott Stop"
+ },
+ {
+ "name": "Yanerones",
+ "pos_x": 24.03125,
+ "pos_y": -124.4375,
+ "pos_z": -86.1875,
+ "stations": "Palmer Installation,Taylor's Folly"
+ },
+ {
+ "name": "Yango",
+ "pos_x": 70.03125,
+ "pos_y": -19.34375,
+ "pos_z": -149.875,
+ "stations": "Butler's Pride"
+ },
+ {
+ "name": "Yankas",
+ "pos_x": 31.90625,
+ "pos_y": -150.40625,
+ "pos_z": 64.84375,
+ "stations": "Aikin Bastion,Kondo Settlement,Regiomontanus Relay"
+ },
+ {
+ "name": "Yankondu",
+ "pos_x": 29.40625,
+ "pos_y": 132.8125,
+ "pos_z": -134.71875,
+ "stations": "Brooks Hub"
+ },
+ {
+ "name": "Yano",
+ "pos_x": -60.03125,
+ "pos_y": 31.1875,
+ "pos_z": -164.375,
+ "stations": "Al Saud Stop,Tryggvason Terminal"
+ },
+ {
+ "name": "Yanoi",
+ "pos_x": 1,
+ "pos_y": -165.5,
+ "pos_z": -94.21875,
+ "stations": "Borrego Outpost"
+ },
+ {
+ "name": "Yanomami",
+ "pos_x": 87.21875,
+ "pos_y": -128.34375,
+ "pos_z": 114.6875,
+ "stations": "Schwarzschild Installation,Cassini Relay,Hevelius Survey,Longomontanus City"
+ },
+ {
+ "name": "Yans",
+ "pos_x": 135.90625,
+ "pos_y": 20.6875,
+ "pos_z": -13.71875,
+ "stations": "Moffitt Outpost,Nicolet Colony"
+ },
+ {
+ "name": "Yansisma",
+ "pos_x": 47.6875,
+ "pos_y": 25.21875,
+ "pos_z": 109.03125,
+ "stations": "Slayton Station,Garriott Enterprise,Borlaug Dock,Taylor Vista"
+ },
+ {
+ "name": "Yantja",
+ "pos_x": 155.03125,
+ "pos_y": 42.46875,
+ "pos_z": -4.0625,
+ "stations": "Temple Works"
+ },
+ {
+ "name": "Yanyamadimo",
+ "pos_x": 120.1875,
+ "pos_y": -123.5625,
+ "pos_z": 152.625,
+ "stations": "Robert Dock,Legendre Barracks"
+ },
+ {
+ "name": "Yanyan",
+ "pos_x": -40.3125,
+ "pos_y": 42.40625,
+ "pos_z": -8.46875,
+ "stations": "Sharipov Ring"
+ },
+ {
+ "name": "Yao Tzu",
+ "pos_x": 82.78125,
+ "pos_y": -174.6875,
+ "pos_z": 28.3125,
+ "stations": "Orbik Port"
+ },
+ {
+ "name": "Yapelici",
+ "pos_x": -77.5625,
+ "pos_y": -103.0625,
+ "pos_z": -18.8125,
+ "stations": "Lonchakov Orbital,Kubasov Station,Popovich Orbital,Wolfe City,Darnielle Relay"
+ },
+ {
+ "name": "Yapep",
+ "pos_x": 90.375,
+ "pos_y": -136.25,
+ "pos_z": -49.0625,
+ "stations": "Herbig Orbital,Pacheco Hub,Bruck Orbital,Severin Terminal,Fernandes Beacon,Nachtigal Penal colony,Pennington Gateway,Arisman Port,Rees City,Hoffman Settlement,Wafer Point"
+ },
+ {
+ "name": "Yapese",
+ "pos_x": -78.59375,
+ "pos_y": -23.71875,
+ "pos_z": -114.03125,
+ "stations": "Fidalgo Colony,Julian Settlement"
+ },
+ {
+ "name": "Yaque",
+ "pos_x": 131.125,
+ "pos_y": -136.65625,
+ "pos_z": 78.875,
+ "stations": "Barnwell Port,Hague Gateway,Stechkin Station"
+ },
+ {
+ "name": "Yaqui",
+ "pos_x": 94.59375,
+ "pos_y": -98.25,
+ "pos_z": 122.28125,
+ "stations": "Shunkai Platform"
+ },
+ {
+ "name": "Yaribon",
+ "pos_x": 11.4375,
+ "pos_y": -121.375,
+ "pos_z": 125.25,
+ "stations": "Yanai Hub,Hay Station,MacVicar Enterprise,Gustav Sporer Dock"
+ },
+ {
+ "name": "Yarica",
+ "pos_x": -162.3125,
+ "pos_y": 10.40625,
+ "pos_z": -8.84375,
+ "stations": "Mitchison Gateway,Cartan Terminal"
+ },
+ {
+ "name": "Yaricans",
+ "pos_x": 87.875,
+ "pos_y": 23.375,
+ "pos_z": -58.40625,
+ "stations": "McMahon Hub,Williams Terminal,Tevis Station,Key Terminal"
+ },
+ {
+ "name": "Yarifukura",
+ "pos_x": 141.65625,
+ "pos_y": -129.625,
+ "pos_z": 5.3125,
+ "stations": "Shklovsky Terminal"
+ },
+ {
+ "name": "Yarigui",
+ "pos_x": -72.5625,
+ "pos_y": 2.5625,
+ "pos_z": -25.84375,
+ "stations": "Eschbach Terminal,Kier Ring,Walker Ring,Salgari Hub,Chomsky Keep,Moore Orbital,Kovalevskaya Terminal,Lem Dock,Hartog Terminal"
+ },
+ {
+ "name": "Yaringu",
+ "pos_x": -57.21875,
+ "pos_y": -23.46875,
+ "pos_z": -82.71875,
+ "stations": "Al Saud City,Furukawa's Folly"
+ },
+ {
+ "name": "Yaro",
+ "pos_x": 131.6875,
+ "pos_y": -11.46875,
+ "pos_z": 26.625,
+ "stations": "Reynolds Horizons,Szameit Mines"
+ },
+ {
+ "name": "Yaroi",
+ "pos_x": 100.8125,
+ "pos_y": 37.40625,
+ "pos_z": -74.9375,
+ "stations": "Borisenko Orbital,Daimler Hub,Nikolayev Dock,Crouch Enterprise,Vaugh Terminal,Frick Orbital,Margulis Station"
+ },
+ {
+ "name": "Yaroklis",
+ "pos_x": 44.78125,
+ "pos_y": -99.5625,
+ "pos_z": 51.40625,
+ "stations": "Naubakht Vision,Marsden City,Drake Station,Graham Station,Lyulka Ring,Otus Gateway,Delany Works,Payson Holdings,Allen St. John Port,Hornoch Prospect"
+ },
+ {
+ "name": "Yarraluk",
+ "pos_x": 131.15625,
+ "pos_y": -240.9375,
+ "pos_z": -23.125,
+ "stations": "Taylor Jr. Survey"
+ },
+ {
+ "name": "Yarram",
+ "pos_x": 67.84375,
+ "pos_y": -73.5,
+ "pos_z": 115.75,
+ "stations": "Leuschner Hangar"
+ },
+ {
+ "name": "Yarrinic",
+ "pos_x": -123.59375,
+ "pos_y": -59.03125,
+ "pos_z": -3.0625,
+ "stations": "Citi Platform"
+ },
+ {
+ "name": "Yarrite",
+ "pos_x": 114,
+ "pos_y": -103.375,
+ "pos_z": 6.34375,
+ "stations": "Deslandres Terminal,Kandrup Dock,Flammarion Refinery,Krylov Depot,Gorey Port"
+ },
+ {
+ "name": "Yarruci",
+ "pos_x": -3.40625,
+ "pos_y": 30.5625,
+ "pos_z": 124.21875,
+ "stations": "Kandel Barracks,Onufriyenko Hub"
+ },
+ {
+ "name": "Yash",
+ "pos_x": -21.84375,
+ "pos_y": -108.3125,
+ "pos_z": 39.25,
+ "stations": "Drebbel Port,Powell Prospect,Gantt Station,Onufrienko Mines"
+ },
+ {
+ "name": "Yashas",
+ "pos_x": -75.90625,
+ "pos_y": -123.21875,
+ "pos_z": -10.09375,
+ "stations": "Schachner Enterprise,Brothers Hub,Baker's Inheritance"
+ },
+ {
+ "name": "Yashi",
+ "pos_x": 121.5,
+ "pos_y": -189.96875,
+ "pos_z": 78.34375,
+ "stations": "Edwards Arena,Wilhelm von Struve Stop"
+ },
+ {
+ "name": "Yashians",
+ "pos_x": 16,
+ "pos_y": 60.78125,
+ "pos_z": -101.4375,
+ "stations": "Berliner Landing,Duffy Dock,Barsanti Terminal"
+ },
+ {
+ "name": "Yaso Kondi",
+ "pos_x": 4.9375,
+ "pos_y": -175.90625,
+ "pos_z": -0.90625,
+ "stations": "Wheeler Market,Mawson Point,Hertzsprung Terminal,Fabricius Vision,Valz Dock,Keeler Port,Jones Orbital,Bessel Port,Robinson Installation,Webb's Inheritance"
+ },
+ {
+ "name": "Yaur",
+ "pos_x": 60.40625,
+ "pos_y": -136.6875,
+ "pos_z": 79.28125,
+ "stations": "Lyot Dock,Edwards Depot"
+ },
+ {
+ "name": "Yaure",
+ "pos_x": 3.3125,
+ "pos_y": -119.3125,
+ "pos_z": 98.6875,
+ "stations": "Schoenherr Outpost"
+ },
+ {
+ "name": "Yauri",
+ "pos_x": 30,
+ "pos_y": -71.75,
+ "pos_z": 85.40625,
+ "stations": "Sewell Terminal,Ryle Dock,Rosse Penal colony,Bracewell Platform"
+ },
+ {
+ "name": "Yaurnai",
+ "pos_x": -99.65625,
+ "pos_y": 116.5625,
+ "pos_z": 43.40625,
+ "stations": "Gerlache Orbital,Lupoff Survey,Steiner Vision,Hugh Reach"
+ },
+ {
+ "name": "Yavapai",
+ "pos_x": -48.75,
+ "pos_y": 9.03125,
+ "pos_z": 90.84375,
+ "stations": "Virtanen Gateway,Coulomb Hub,Wohler Orbital"
+ },
+ {
+ "name": "Yavas",
+ "pos_x": 73.3125,
+ "pos_y": 94.96875,
+ "pos_z": 27.84375,
+ "stations": "Bisson Dock,Tennyson d'Eyncourt Dock,Klein Hub"
+ },
+ {
+ "name": "Yavatas",
+ "pos_x": 175.5,
+ "pos_y": -144.96875,
+ "pos_z": 23.90625,
+ "stations": "Westerhout Port"
+ },
+ {
+ "name": "Yawa",
+ "pos_x": 54.65625,
+ "pos_y": -86.125,
+ "pos_z": 23.4375,
+ "stations": "Dean Hub"
+ },
+ {
+ "name": "Yawal",
+ "pos_x": -44.0625,
+ "pos_y": -58.21875,
+ "pos_z": 76.1875,
+ "stations": "Levy Retreat,Pohl's Pride"
+ },
+ {
+ "name": "Yawala",
+ "pos_x": -4.09375,
+ "pos_y": -15.34375,
+ "pos_z": -100.125,
+ "stations": "Szebehely Settlement,Bayliss Outpost"
+ },
+ {
+ "name": "Yawalapiti",
+ "pos_x": 203.75,
+ "pos_y": -173.75,
+ "pos_z": 68.125,
+ "stations": "Brosnatch Vision,Whipple's Progress,Cartan Reach"
+ },
+ {
+ "name": "Yax Balam",
+ "pos_x": -23.53125,
+ "pos_y": -22.5625,
+ "pos_z": -95.34375,
+ "stations": "Ivanchenkov Plant,Kendrick Holdings"
+ },
+ {
+ "name": "Ye'kua'gsa",
+ "pos_x": 36.53125,
+ "pos_y": -23.65625,
+ "pos_z": 58.46875,
+ "stations": "Higginbotham Mines,Ehrlich Landing"
+ },
+ {
+ "name": "Ye'kuana",
+ "pos_x": 32.46875,
+ "pos_y": -99.875,
+ "pos_z": -84.46875,
+ "stations": "Herzfeld Orbital,Herschel Dock,Howard Station,Joliot-Curie City,Kinsey Terminal,Alvares Vision,Roberts City,Birkeland Vision,Parise Station,Carrier Ring"
+ },
+ {
+ "name": "Ye'kuape",
+ "pos_x": 31.5625,
+ "pos_y": -15.8125,
+ "pos_z": 58.1875,
+ "stations": "Gauss Dock,Bobko Hub,Strzelecki Prospect"
+ },
+ {
+ "name": "Ye'kuapemas",
+ "pos_x": -70.15625,
+ "pos_y": 44.625,
+ "pos_z": -33.65625,
+ "stations": "Kekule Orbital,Ryman Base"
+ },
+ {
+ "name": "Yebatayu",
+ "pos_x": -135.1875,
+ "pos_y": -40.5,
+ "pos_z": 7.40625,
+ "stations": "Skolem Colony,Bradshaw's Progress"
+ },
+ {
+ "name": "Yebayo",
+ "pos_x": 18.25,
+ "pos_y": 105.21875,
+ "pos_z": -34.875,
+ "stations": "Barnaby Survey,Amundsen Outpost,Carpini Base"
+ },
+ {
+ "name": "Yed Prior",
+ "pos_x": -23.25,
+ "pos_y": 89.625,
+ "pos_z": 143.875,
+ "stations": "Sylvester Bastion"
+ },
+ {
+ "name": "Yemai",
+ "pos_x": -125.34375,
+ "pos_y": 114.03125,
+ "pos_z": 51.21875,
+ "stations": "Gibson Terminal,Krylov Arsenal,Bottego Horizons,Neville Landing"
+ },
+ {
+ "name": "Yemaia",
+ "pos_x": -105.59375,
+ "pos_y": -11.21875,
+ "pos_z": -28.0625,
+ "stations": "Hartog Relay,Doyle Asylum"
+ },
+ {
+ "name": "Yemairwar",
+ "pos_x": 60.96875,
+ "pos_y": 67.90625,
+ "pos_z": 84.8125,
+ "stations": "Haldeman II Outpost,Fu Point"
+ },
+ {
+ "name": "Yemaki",
+ "pos_x": -91.15625,
+ "pos_y": 16.90625,
+ "pos_z": -89.84375,
+ "stations": "Gidzenko Hangar,Burbank Terminal"
+ },
+ {
+ "name": "Yemakinni",
+ "pos_x": -23.15625,
+ "pos_y": -78.78125,
+ "pos_z": -140.03125,
+ "stations": "Chaviano Colony,Fibonacci Camp"
+ },
+ {
+ "name": "Yemandegou",
+ "pos_x": -22.46875,
+ "pos_y": -147.28125,
+ "pos_z": 72.71875,
+ "stations": "McDermott Port"
+ },
+ {
+ "name": "Yemanto",
+ "pos_x": -94.53125,
+ "pos_y": 103.5625,
+ "pos_z": -43.71875,
+ "stations": "Treshchov Dock"
+ },
+ {
+ "name": "Yemar",
+ "pos_x": 167.96875,
+ "pos_y": 23.875,
+ "pos_z": 2.5625,
+ "stations": "Usachov Terminal"
+ },
+ {
+ "name": "Yembo",
+ "pos_x": -97.40625,
+ "pos_y": -10.125,
+ "pos_z": 49.8125,
+ "stations": "Naddoddur Terminal,Mitra Vision,Lichtenberg Terminal,Litke Reach,Behring's Folly"
+ },
+ {
+ "name": "Yemojong Ku",
+ "pos_x": 75.59375,
+ "pos_y": -156,
+ "pos_z": -74.6875,
+ "stations": "Watson Landing,Kanai Port"
+ },
+ {
+ "name": "Yemotepa",
+ "pos_x": -120.125,
+ "pos_y": 52.875,
+ "pos_z": -40.4375,
+ "stations": "Everest Vision,Farkas Works,Lopez-Alegria Base"
+ },
+ {
+ "name": "Yemotwa",
+ "pos_x": 38.09375,
+ "pos_y": -217.5,
+ "pos_z": 64.8125,
+ "stations": "Kerimov Survey,Maury Beacon"
+ },
+ {
+ "name": "Yemovicenuo",
+ "pos_x": 104.28125,
+ "pos_y": -61.21875,
+ "pos_z": -54.21875,
+ "stations": "Jones Orbital,Keyes Horizons,Jones Legacy"
+ },
+ {
+ "name": "Yen Camus",
+ "pos_x": 145.46875,
+ "pos_y": 61.3125,
+ "pos_z": -73.5,
+ "stations": "Maudslay Platform,Hertz City,Vishweswarayya Ring,Rochon Landing,Scheerbart Beacon"
+ },
+ {
+ "name": "Yen Chelici",
+ "pos_x": -188.15625,
+ "pos_y": -49.28125,
+ "pos_z": -43.71875,
+ "stations": "Wallace Hub,Holland Orbital,Slonczewski Dock,Coney Holdings"
+ },
+ {
+ "name": "Yen Di",
+ "pos_x": 4.78125,
+ "pos_y": -44.09375,
+ "pos_z": -92.84375,
+ "stations": "Crown Dock"
+ },
+ {
+ "name": "Yen Ek",
+ "pos_x": 123.78125,
+ "pos_y": -77.75,
+ "pos_z": -34.96875,
+ "stations": "Champlain Barracks,Foerster Vision,Tanaka Vision,Balog Landing"
+ },
+ {
+ "name": "Yen Hsin",
+ "pos_x": 66.5625,
+ "pos_y": -48.84375,
+ "pos_z": 59.78125,
+ "stations": "Haipeng Depot"
+ },
+ {
+ "name": "Yen Hsini",
+ "pos_x": 82.84375,
+ "pos_y": -210.03125,
+ "pos_z": 52.59375,
+ "stations": "Chaffee Vision,Kidinnu Penal colony,Koishikawa Platform"
+ },
+ {
+ "name": "Yen K'an",
+ "pos_x": -90.375,
+ "pos_y": 2.4375,
+ "pos_z": -179.75,
+ "stations": "Griffiths' Progress"
+ },
+ {
+ "name": "Yen K'uie",
+ "pos_x": 87,
+ "pos_y": -89.75,
+ "pos_z": -54.3125,
+ "stations": "Siddha Gateway,Escobar Lab"
+ },
+ {
+ "name": "Yen Ku",
+ "pos_x": 72.71875,
+ "pos_y": -255.71875,
+ "pos_z": 33.1875,
+ "stations": "Fernandes Silo,Dalgarno Hub"
+ },
+ {
+ "name": "Yen Kuikul",
+ "pos_x": 122.375,
+ "pos_y": -95.84375,
+ "pos_z": -37.78125,
+ "stations": "Ulloa Terminal,Bothezat Beacon"
+ },
+ {
+ "name": "Yen Maniama",
+ "pos_x": 60.40625,
+ "pos_y": -3.15625,
+ "pos_z": 156.21875,
+ "stations": "Eschbach Platform,Blaylock Enterprise"
+ },
+ {
+ "name": "Yen Nu",
+ "pos_x": 22.84375,
+ "pos_y": -130.0625,
+ "pos_z": -87.625,
+ "stations": "Hermite Port,Clauss Hub,Stjepan Seljan Dock,Heinlein Beacon,Walters' Progress"
+ },
+ {
+ "name": "Yen Shesmu",
+ "pos_x": -118.125,
+ "pos_y": 99.8125,
+ "pos_z": 26.59375,
+ "stations": "Cartmill Estate"
+ },
+ {
+ "name": "Yen Tacanik",
+ "pos_x": 207.625,
+ "pos_y": -174.84375,
+ "pos_z": 49.625,
+ "stations": "Sewell Port,Hind Terminal,Mather Point"
+ },
+ {
+ "name": "Yen Ti",
+ "pos_x": -55.5625,
+ "pos_y": -24.875,
+ "pos_z": -26.34375,
+ "stations": "Pordenone Vision,Samuda Plant,Barbosa Colony,Ford Plant"
+ },
+ {
+ "name": "Yena Gu",
+ "pos_x": -86.625,
+ "pos_y": -121.75,
+ "pos_z": 63.34375,
+ "stations": "Litke Terminal,Lindbohm Settlement,Sarrantonio Survey,Rand's Folly"
+ },
+ {
+ "name": "Yenada",
+ "pos_x": 60.34375,
+ "pos_y": -20.90625,
+ "pos_z": 44.53125,
+ "stations": "Stafford Landing,Piccard Settlement"
+ },
+ {
+ "name": "Yenasaki",
+ "pos_x": -97.875,
+ "pos_y": -71.625,
+ "pos_z": -113.15625,
+ "stations": "Ostwald Stop,Sauma Penitentiary"
+ },
+ {
+ "name": "Yenates",
+ "pos_x": 44.34375,
+ "pos_y": 30.40625,
+ "pos_z": 79.90625,
+ "stations": "Ashton Port"
+ },
+ {
+ "name": "Yeng",
+ "pos_x": 156.375,
+ "pos_y": -98.625,
+ "pos_z": -20.5,
+ "stations": "Ryan City,Ghez Landing,Shirazi Vision"
+ },
+ {
+ "name": "Yenic",
+ "pos_x": 126.90625,
+ "pos_y": 41.625,
+ "pos_z": 28.65625,
+ "stations": "Walters Dock,Sylvester Dock,Asimov Dock,Stephenson Survey,Meyrink Arsenal"
+ },
+ {
+ "name": "Yenici",
+ "pos_x": 120,
+ "pos_y": -119.5,
+ "pos_z": -3.0625,
+ "stations": "Lloyd Wright Terminal,Olahus Station,Mozhaysky Vision,Ahnert-Rohlfs Orbital,Faye Hub,Paola Hub,Wood Works,Dedekind Depot,Harvey-Smith Hub,Nicollet Enterprise"
+ },
+ {
+ "name": "Yeniklis",
+ "pos_x": -19.0625,
+ "pos_y": -64.28125,
+ "pos_z": 30.1875,
+ "stations": "Kornbluth City,Bunch Prospect"
+ },
+ {
+ "name": "Yeninuri",
+ "pos_x": -139.28125,
+ "pos_y": 24.59375,
+ "pos_z": 77.5625,
+ "stations": "Khan Colony,Walter Relay,Feustel Enterprise,Besonders Beacon"
+ },
+ {
+ "name": "Yenis",
+ "pos_x": 147.84375,
+ "pos_y": -140.96875,
+ "pos_z": -13.6875,
+ "stations": "Louis de Lacaille Beacon,Sheffield Vision"
+ },
+ {
+ "name": "Yenisei",
+ "pos_x": 71.375,
+ "pos_y": -164.75,
+ "pos_z": 104.96875,
+ "stations": "Riccioli Hub,Druillet Colony"
+ },
+ {
+ "name": "Yenistani",
+ "pos_x": 109.125,
+ "pos_y": -62.1875,
+ "pos_z": 28.65625,
+ "stations": "Roe Plant,Johri Terminal,Gustafsson Gateway,Miller Port,Asprin's Claim"
+ },
+ {
+ "name": "Yer Tanri",
+ "pos_x": 2.875,
+ "pos_y": -47.65625,
+ "pos_z": 54.1875,
+ "stations": "Tshang Platform,May's Claim"
+ },
+ {
+ "name": "Yera",
+ "pos_x": 65.21875,
+ "pos_y": -27.1875,
+ "pos_z": -47.21875,
+ "stations": "Garn Stop"
+ },
+ {
+ "name": "Yeraeang",
+ "pos_x": 113.375,
+ "pos_y": 22.5,
+ "pos_z": 114.34375,
+ "stations": "d'Eyncourt Base,Grzimek Dock,Viete Landing"
+ },
+ {
+ "name": "Yerava",
+ "pos_x": -55.34375,
+ "pos_y": -53.875,
+ "pos_z": -72.34375,
+ "stations": "Levinson Refinery,Hughes Camp"
+ },
+ {
+ "name": "Yggdrajang",
+ "pos_x": 28.3125,
+ "pos_y": 0.84375,
+ "pos_z": -67.875,
+ "stations": "Selberg's Inheritance,Laumer's Progress,Schade Platform"
+ },
+ {
+ "name": "Yhele",
+ "pos_x": 2.1875,
+ "pos_y": -151.21875,
+ "pos_z": 97.53125,
+ "stations": "McNaught Dock,Balog Terminal,Xin Dock,Selous Enterprise,Wendelin Enterprise,Baldwin Depot"
+ },
+ {
+ "name": "Yheling",
+ "pos_x": -9.65625,
+ "pos_y": 129.96875,
+ "pos_z": 106.5625,
+ "stations": "Matheson City,Shaikh Gateway,Artin Ring,Shosuke Enterprise,Wallace Depot"
+ },
+ {
+ "name": "Yi Dhodere",
+ "pos_x": -114,
+ "pos_y": 44.6875,
+ "pos_z": 83.03125,
+ "stations": "Vasquez de Coronado Works,Nixon Settlement,Rubruck Depot"
+ },
+ {
+ "name": "Yi Trica",
+ "pos_x": -117.5,
+ "pos_y": 70.59375,
+ "pos_z": -82.125,
+ "stations": "Tshang Vision,Pierres Gateway,Neumann Lab,Humphreys Terminal"
+ },
+ {
+ "name": "Yimakuapa",
+ "pos_x": -30.3125,
+ "pos_y": -49.375,
+ "pos_z": -71.09375,
+ "stations": "Frobisher Terminal,Elgin Port,Marques Gateway,Brothers Vision"
+ },
+ {
+ "name": "Yiman",
+ "pos_x": -83.96875,
+ "pos_y": -95.46875,
+ "pos_z": 74,
+ "stations": "Macgregor Colony,Piccard Outpost"
+ },
+ {
+ "name": "Yimanbin",
+ "pos_x": 51.40625,
+ "pos_y": -51.34375,
+ "pos_z": 37.03125,
+ "stations": "Worden Gateway,Lindsey Hub,Farkas Port,Vaucanson Gateway,Knight Gateway,Mach City,Hawley Dock,Bridges Port,Clair Beacon,Rosenberg Penal colony,Afanasyev Gateway,Poleshchuk Horizons,Crowley Depot"
+ },
+ {
+ "name": "Yimash",
+ "pos_x": 128.34375,
+ "pos_y": 33.84375,
+ "pos_z": 49.34375,
+ "stations": "Glashow Settlement,Deluc Port,Rennie Orbital"
+ },
+ {
+ "name": "Yin Shaka",
+ "pos_x": 61.8125,
+ "pos_y": 19.28125,
+ "pos_z": -146.0625,
+ "stations": "McKee Ring,Mitchell Horizons,Herbert Hub"
+ },
+ {
+ "name": "Yin T'ien",
+ "pos_x": -50.84375,
+ "pos_y": -67.25,
+ "pos_z": -22.78125,
+ "stations": "Pierce Port"
+ },
+ {
+ "name": "Yin Yin",
+ "pos_x": 67.78125,
+ "pos_y": 129.75,
+ "pos_z": -34.625,
+ "stations": "MacLean Freeport"
+ },
+ {
+ "name": "Yinegafo",
+ "pos_x": 19.84375,
+ "pos_y": -203.59375,
+ "pos_z": -20.90625,
+ "stations": "Pollas Port,Wafa Horizons"
+ },
+ {
+ "name": "Yinered",
+ "pos_x": 26.6875,
+ "pos_y": -33.46875,
+ "pos_z": -160.03125,
+ "stations": "Morukov Port"
+ },
+ {
+ "name": "Yinesheimr",
+ "pos_x": 151.03125,
+ "pos_y": -122.71875,
+ "pos_z": 20.46875,
+ "stations": "Gurney Works,Miller's Inheritance"
+ },
+ {
+ "name": "Yingites",
+ "pos_x": 145.53125,
+ "pos_y": -51.25,
+ "pos_z": -30.71875,
+ "stations": "Naddoddur Dock"
+ },
+ {
+ "name": "Yingo",
+ "pos_x": -84.875,
+ "pos_y": -43.21875,
+ "pos_z": -14.53125,
+ "stations": "Onufriyenko Hub,Pippin Enterprise,Wisoff Keep,Cormack Port"
+ },
+ {
+ "name": "Yinjian",
+ "pos_x": 26.8125,
+ "pos_y": -34.875,
+ "pos_z": -5.875,
+ "stations": "H-Man's Hub,June's Folly,Plante Enterprise,Norgay's Pride,Utley's Pride"
+ },
+ {
+ "name": "Ymbe",
+ "pos_x": 52.21875,
+ "pos_y": -52.90625,
+ "pos_z": 146.15625,
+ "stations": "Hussenot Port,Burnham Hub,Babcock Relay"
+ },
+ {
+ "name": "Yngvir",
+ "pos_x": -148.09375,
+ "pos_y": 33.96875,
+ "pos_z": 66,
+ "stations": "de Andrade Platform,Parry Port,Whymper Horizons"
+ },
+ {
+ "name": "Yokudi",
+ "pos_x": -23.59375,
+ "pos_y": 8.96875,
+ "pos_z": 111.8125,
+ "stations": "Hoften Orbital"
+ },
+ {
+ "name": "Yokusu",
+ "pos_x": 24.46875,
+ "pos_y": -188,
+ "pos_z": -19.75,
+ "stations": "Edwards Port,Barr Landing,Kirk Depot,Fedden's Inheritance,Mawson Base"
+ },
+ {
+ "name": "Yolngikapu",
+ "pos_x": 168.6875,
+ "pos_y": -53.875,
+ "pos_z": 31.15625,
+ "stations": "Lenthall Stop,Willis Depot,Nansen Stop"
+ },
+ {
+ "name": "Yorates",
+ "pos_x": 60.34375,
+ "pos_y": 17.875,
+ "pos_z": 59.65625,
+ "stations": "Zalyotin Dock,Lichtenberg Gateway,Wyeth Legacy,Babbage Gateway,Tesla Beacon"
+ },
+ {
+ "name": "Yoru",
+ "pos_x": 97.875,
+ "pos_y": -86.90625,
+ "pos_z": 64.125,
+ "stations": "Nemo Cyber Party Base,Manning Base,Danforth Prospect,Caidin Reach"
+ },
+ {
+ "name": "Yorua",
+ "pos_x": -7.40625,
+ "pos_y": -9.78125,
+ "pos_z": 76.1875,
+ "stations": "Forrester Station,Blackwell Hub,Hassanein Installation,Rennie Hub"
+ },
+ {
+ "name": "Yoruba",
+ "pos_x": -46,
+ "pos_y": 35.84375,
+ "pos_z": -34.21875,
+ "stations": "Apt Orbital,Atkov Station,Carpenter Terminal,Coleman Enterprise,Mendez Dock,Balandin Ring,Nikolayev Dock,Newcomen Landing,Resnick Relay,Irvin Gateway,Bosch Relay"
+ },
+ {
+ "name": "Yorundi",
+ "pos_x": 88.375,
+ "pos_y": 35.34375,
+ "pos_z": -9.34375,
+ "stations": "Fabian Vision,Archimedes Landing"
+ },
+ {
+ "name": "Yota",
+ "pos_x": 80.71875,
+ "pos_y": -51.59375,
+ "pos_z": 24.3125,
+ "stations": "Favier Settlement,Baturin Vision"
+ },
+ {
+ "name": "Yotani",
+ "pos_x": -83.59375,
+ "pos_y": -80.0625,
+ "pos_z": 72.6875,
+ "stations": "Fermi Escape,Godwin Landing"
+ },
+ {
+ "name": "Youming",
+ "pos_x": -15.28125,
+ "pos_y": -33.4375,
+ "pos_z": 25.84375,
+ "stations": "Pike's Progress,Warner Mines,Bierce Station"
+ },
+ {
+ "name": "Ys",
+ "pos_x": 70.21875,
+ "pos_y": -137.40625,
+ "pos_z": 63.6875,
+ "stations": "Ender's Dream,Ashdown,von Biela Settlement,Al Sufi Landing"
+ },
+ {
+ "name": "Yu Ch'iang",
+ "pos_x": -79.625,
+ "pos_y": 92.78125,
+ "pos_z": 100.375,
+ "stations": "Vesalius Survey,Conrad Terminal,Asimov Keep"
+ },
+ {
+ "name": "Yu Chiko Oh",
+ "pos_x": 55.28125,
+ "pos_y": -42.59375,
+ "pos_z": -102.25,
+ "stations": "Issigonis Terminal"
+ },
+ {
+ "name": "Yu Chuj",
+ "pos_x": 46.0625,
+ "pos_y": -163.59375,
+ "pos_z": 69.125,
+ "stations": "Dyson Dock,Severin Ring"
+ },
+ {
+ "name": "Yu Di Wandy",
+ "pos_x": 73.15625,
+ "pos_y": 133.75,
+ "pos_z": -39.46875,
+ "stations": "Martins Asylum,Neff Landing"
+ },
+ {
+ "name": "Yu Di Yi",
+ "pos_x": -86.65625,
+ "pos_y": -3.875,
+ "pos_z": 47.5,
+ "stations": "Aksyonov Orbital,Stewart's Inheritance,Gibson Colony"
+ },
+ {
+ "name": "Yu Diegus",
+ "pos_x": 99.6875,
+ "pos_y": -112.125,
+ "pos_z": 37.28125,
+ "stations": "Gorey Landing"
+ },
+ {
+ "name": "Yu Hai",
+ "pos_x": -99.5,
+ "pos_y": 80.0625,
+ "pos_z": -100.59375,
+ "stations": "Lee Mines"
+ },
+ {
+ "name": "Yu Hsien Tu",
+ "pos_x": -55.34375,
+ "pos_y": 105.21875,
+ "pos_z": 14.875,
+ "stations": "Mackenzie Terminal,Szentmartony Orbital,Everest Laboratory,Hendel Holdings,Kippax Penal colony,Norgay Ring,Acharya Terminal,Kennedy Ring"
+ },
+ {
+ "name": "Yu Hsinda",
+ "pos_x": 81.09375,
+ "pos_y": -196.53125,
+ "pos_z": 40.6875,
+ "stations": "Jenkins Camp,Smith Depot"
+ },
+ {
+ "name": "Yu Kop",
+ "pos_x": -24.4375,
+ "pos_y": 68.96875,
+ "pos_z": 111.625,
+ "stations": "Szebehely Survey,Hernandez Beacon,Volterra Base"
+ },
+ {
+ "name": "Yu Kuo Wang",
+ "pos_x": 105.4375,
+ "pos_y": -9.78125,
+ "pos_z": -114.9375,
+ "stations": "Parsons Outpost,Fischer Mines,Galois Refinery"
+ },
+ {
+ "name": "Yu Qiano",
+ "pos_x": 78.875,
+ "pos_y": 148.4375,
+ "pos_z": 17.625,
+ "stations": "Ore Works"
+ },
+ {
+ "name": "Yu Shanwen",
+ "pos_x": 190.40625,
+ "pos_y": -115.21875,
+ "pos_z": -2.53125,
+ "stations": "Lagadha Arena"
+ },
+ {
+ "name": "Yu Shedi",
+ "pos_x": 84.96875,
+ "pos_y": 111.625,
+ "pos_z": 6.28125,
+ "stations": "Wegener Ring,Varthema Bastion"
+ },
+ {
+ "name": "Yu Shonsa",
+ "pos_x": 161.5625,
+ "pos_y": -180.46875,
+ "pos_z": -22.53125,
+ "stations": "Kidinnu Point,Peary Enterprise"
+ },
+ {
+ "name": "Yu Shor",
+ "pos_x": 126.6875,
+ "pos_y": -160.6875,
+ "pos_z": -21.78125,
+ "stations": "Bus Stop"
+ },
+ {
+ "name": "Yu Shou",
+ "pos_x": 60.9375,
+ "pos_y": -64.3125,
+ "pos_z": -38.78125,
+ "stations": "Vuia Landing,Carrington Station,Bahcall Refinery,Puleston Hub"
+ },
+ {
+ "name": "Yu Shun",
+ "pos_x": 171.71875,
+ "pos_y": -109.875,
+ "pos_z": -20.71875,
+ "stations": "Sheepshanks Dock,Galindo Prospect,Schoening Hub,Argelander Ring"
+ },
+ {
+ "name": "Yu Song Di",
+ "pos_x": 154.03125,
+ "pos_y": -7,
+ "pos_z": 58.21875,
+ "stations": "Weitz Enterprise,Bondar Enterprise,Stabenow Terminal"
+ },
+ {
+ "name": "Yu Ti Wand",
+ "pos_x": -142.75,
+ "pos_y": -33.46875,
+ "pos_z": -83.5625,
+ "stations": "Al-Farabi Orbital,Payton Terminal"
+ },
+ {
+ "name": "Yu Tiku",
+ "pos_x": -1.71875,
+ "pos_y": -38.5625,
+ "pos_z": 87.90625,
+ "stations": "McKee Station,Lucid's Inheritance,Surayev Point"
+ },
+ {
+ "name": "Yu Tikua",
+ "pos_x": -2.1875,
+ "pos_y": 66.71875,
+ "pos_z": 98.71875,
+ "stations": "Thorne Outpost"
+ },
+ {
+ "name": "Yu Tun",
+ "pos_x": 104.71875,
+ "pos_y": -12.875,
+ "pos_z": -32.25,
+ "stations": "Dall Reach"
+ },
+ {
+ "name": "Yucadros",
+ "pos_x": -136.1875,
+ "pos_y": 22.75,
+ "pos_z": 77.0625,
+ "stations": "Dana Platform,Irwin Enterprise,Baudin Beacon,Laird Refinery"
+ },
+ {
+ "name": "Yucan",
+ "pos_x": -54.375,
+ "pos_y": 59.5,
+ "pos_z": -81.28125,
+ "stations": "Khrunov Orbital,Boswell Station,Oleskiw Enterprise,Grzimek Prospect,Hamilton Observatory,Chilton Vision,Merchiston Base"
+ },
+ {
+ "name": "Yucanes",
+ "pos_x": -117.84375,
+ "pos_y": -107.03125,
+ "pos_z": -24.1875,
+ "stations": "Dampier Relay,Pond Outpost"
+ },
+ {
+ "name": "Yudhis",
+ "pos_x": -129.59375,
+ "pos_y": 66,
+ "pos_z": 97.90625,
+ "stations": "Cobb Installation,Kotzebue's Claim,Fernandes de Queiros Enterprise"
+ },
+ {
+ "name": "Yudhriges",
+ "pos_x": 53.40625,
+ "pos_y": 76.6875,
+ "pos_z": 129.65625,
+ "stations": "Vercors Orbital,Tapinas Orbital"
+ },
+ {
+ "name": "Yudhumij",
+ "pos_x": 6.875,
+ "pos_y": -89.96875,
+ "pos_z": 127.21875,
+ "stations": "Phillips Depot,Zaschka City,Osterbrock Ring"
+ },
+ {
+ "name": "Yudhumit",
+ "pos_x": -134.15625,
+ "pos_y": -68.3125,
+ "pos_z": 25.28125,
+ "stations": "Macquorn Rankine Holdings,Jernigan Dock"
+ },
+ {
+ "name": "Yuets",
+ "pos_x": 157,
+ "pos_y": -112.6875,
+ "pos_z": 109.5625,
+ "stations": "Fabricius Hub,Jenkins Settlement,MacLeod's Claim"
+ },
+ {
+ "name": "Yuetu",
+ "pos_x": 169.5,
+ "pos_y": 37.09375,
+ "pos_z": 39.4375,
+ "stations": "Blenkinsop Vision,Westerfeld Depot,Molina Colony,Reis Installation"
+ },
+ {
+ "name": "Yuetuh Emis",
+ "pos_x": -54.75,
+ "pos_y": 183.625,
+ "pos_z": -33.34375,
+ "stations": "Thompson Orbital,Christopher Enterprise"
+ },
+ {
+ "name": "Yuggera",
+ "pos_x": 92.53125,
+ "pos_y": -101.0625,
+ "pos_z": -47.09375,
+ "stations": "Oikawa Vision,Preuss Laboratory,Flammarion Platform,Offutt Prospect,Lindblad Port"
+ },
+ {
+ "name": "Yuggeri",
+ "pos_x": 59.4375,
+ "pos_y": -98.21875,
+ "pos_z": 144.25,
+ "stations": "Guth Enterprise,Kemurdzhian Horizons,Bigourdan's Inheritance,Starzl Landing"
+ },
+ {
+ "name": "Yuggerians",
+ "pos_x": -77.65625,
+ "pos_y": 152.96875,
+ "pos_z": 35.5625,
+ "stations": "Stairs Outpost,Brin Settlement,Stiegler Base"
+ },
+ {
+ "name": "Yugur",
+ "pos_x": 129.59375,
+ "pos_y": -133.03125,
+ "pos_z": -80.78125,
+ "stations": "Reid Landing"
+ },
+ {
+ "name": "Yuinni",
+ "pos_x": -1.46875,
+ "pos_y": -133.4375,
+ "pos_z": 123.4375,
+ "stations": "Gent Platform,Purbach Platform,Gottlob Frege Vision"
+ },
+ {
+ "name": "Yukait",
+ "pos_x": 164.34375,
+ "pos_y": -64.53125,
+ "pos_z": -34.53125,
+ "stations": "Hale Port,Lassell Plant,Lippisch Survey"
+ },
+ {
+ "name": "Yukan",
+ "pos_x": 77.21875,
+ "pos_y": -104.03125,
+ "pos_z": 81.625,
+ "stations": "Hardy Vision,Matthews Hub,Whipple Vision,Lowell Barracks"
+ },
+ {
+ "name": "Yukas",
+ "pos_x": 116.78125,
+ "pos_y": -114.40625,
+ "pos_z": -38.0625,
+ "stations": "Mattei Station,Hansen Dock,Fermat Reach"
+ },
+ {
+ "name": "Yuki Tiana",
+ "pos_x": -154.15625,
+ "pos_y": 1.03125,
+ "pos_z": 19.46875,
+ "stations": "Hewish Hangar,Phillips Dock,Wylie Base"
+ },
+ {
+ "name": "Yukilam",
+ "pos_x": 93.40625,
+ "pos_y": -235.96875,
+ "pos_z": -25.84375,
+ "stations": "Orbik Terminal,Very Landing,Nehsi Silo,Ferguson Plant"
+ },
+ {
+ "name": "Yukou",
+ "pos_x": -47.875,
+ "pos_y": -74.34375,
+ "pos_z": 90.375,
+ "stations": "Fourier Dock,Sawyer Beacon,Carpini Enterprise"
+ },
+ {
+ "name": "Yukoukha",
+ "pos_x": -134.5625,
+ "pos_y": -34.03125,
+ "pos_z": -4.75,
+ "stations": "Peral Relay,Pook's Progress,Elder Beacon"
+ },
+ {
+ "name": "Yukpeng",
+ "pos_x": 137.75,
+ "pos_y": 23.71875,
+ "pos_z": 79.8125,
+ "stations": "Oppenheimer Platform,Hurston Dock,Ockels Platform,Beekman Vision"
+ },
+ {
+ "name": "Yukpenon",
+ "pos_x": 10.40625,
+ "pos_y": 4.0625,
+ "pos_z": -132.4375,
+ "stations": "Stokes Enterprise,Sekowski Dock,Hill Platform,Glashow Holdings,Webb Beacon"
+ },
+ {
+ "name": "Yulandh",
+ "pos_x": 52.78125,
+ "pos_y": -0.375,
+ "pos_z": 136.0625,
+ "stations": "Berners-Lee City,Brand Point,Howe Park,Kooi Orbital"
+ },
+ {
+ "name": "Yulashis",
+ "pos_x": 190.0625,
+ "pos_y": -170.40625,
+ "pos_z": 30.4375,
+ "stations": "Schoening Dock,Stechkin Prospect,Hall Port,Comer's Inheritance,Hausdorff Refinery"
+ },
+ {
+ "name": "Yum Chayuu",
+ "pos_x": -14.65625,
+ "pos_y": 34.3125,
+ "pos_z": 135.96875,
+ "stations": "Grothendieck Mine"
+ },
+ {
+ "name": "Yum Cimil",
+ "pos_x": 37.28125,
+ "pos_y": -18.59375,
+ "pos_z": -166,
+ "stations": "Acaba Hub,Leslie Terminal,Oefelein Terminal,Alten Installation"
+ },
+ {
+ "name": "Yum Cimmeri",
+ "pos_x": -81.03125,
+ "pos_y": -99.71875,
+ "pos_z": -47.6875,
+ "stations": "Laird's Exile"
+ },
+ {
+ "name": "Yum Cruang",
+ "pos_x": -54.0625,
+ "pos_y": -4.90625,
+ "pos_z": 115.0625,
+ "stations": "Khan Terminal,Aristotle City,Hawking Port,Tsunenaga Installation,Lu Base"
+ },
+ {
+ "name": "Yum Kachan",
+ "pos_x": 129.34375,
+ "pos_y": -24.53125,
+ "pos_z": -73.28125,
+ "stations": "Simonyi Relay,Chilton Depot"
+ },
+ {
+ "name": "Yum Kacoc",
+ "pos_x": 105.75,
+ "pos_y": -96.78125,
+ "pos_z": 131.34375,
+ "stations": "Biruni Orbital,Balog Terminal,Firsoff Hub,Oramus Refinery"
+ },
+ {
+ "name": "Yum Kaia",
+ "pos_x": -40.40625,
+ "pos_y": -76.09375,
+ "pos_z": 19.6875,
+ "stations": "Apgar Orbital,Gamow Station,Phillips Holdings,Resnik Settlement"
+ },
+ {
+ "name": "Yum Kaka",
+ "pos_x": -75.125,
+ "pos_y": 49.6875,
+ "pos_z": -113.90625,
+ "stations": "Rice Port,Harrison Platform,Dayuan Prospect"
+ },
+ {
+ "name": "Yum Kamcabi",
+ "pos_x": 35.84375,
+ "pos_y": 97.65625,
+ "pos_z": -14.21875,
+ "stations": "Dirichlet Orbital,Holub Hub,Keyes Settlement,The Harmony,Greenleaf's Inheritance"
+ },
+ {
+ "name": "Yum Kicha",
+ "pos_x": -42.53125,
+ "pos_y": 85.25,
+ "pos_z": 70.78125,
+ "stations": "Fermi Colony"
+ },
+ {
+ "name": "Yun Dun",
+ "pos_x": -29.5,
+ "pos_y": 49.3125,
+ "pos_z": -116.34375,
+ "stations": "Andrews Hub,Fiennes Dock,Lefschetz Gateway,Crook City,Zetford Hub,Varthema Port,Rothfuss Station"
+ },
+ {
+ "name": "Yun Dzacae",
+ "pos_x": 129,
+ "pos_y": 52.4375,
+ "pos_z": 69.0625,
+ "stations": "Shaver Mines"
+ },
+ {
+ "name": "Yun Hsie",
+ "pos_x": -70.5,
+ "pos_y": -26.59375,
+ "pos_z": 97.9375,
+ "stations": "Felice City"
+ },
+ {
+ "name": "Yun Nigh",
+ "pos_x": -108.96875,
+ "pos_y": 7.71875,
+ "pos_z": -135.90625,
+ "stations": "Binnie Vision,Leestma Works"
+ },
+ {
+ "name": "Yun Piri",
+ "pos_x": 113,
+ "pos_y": 108.4375,
+ "pos_z": -84.28125,
+ "stations": "von Bellingshausen Landing,Davis Beacon,Clapperton Vision"
+ },
+ {
+ "name": "Yun Puggera",
+ "pos_x": -14.59375,
+ "pos_y": -124.71875,
+ "pos_z": 13.3125,
+ "stations": "Hay Terminal"
+ },
+ {
+ "name": "Yun Tzu",
+ "pos_x": 19.96875,
+ "pos_y": -206.34375,
+ "pos_z": 89.5,
+ "stations": "Freas Station,Marcy Ring,Gunter Prospect,Besonders Installation,Danvers Arsenal"
+ },
+ {
+ "name": "Yupini",
+ "pos_x": 89.96875,
+ "pos_y": -201.15625,
+ "pos_z": 24.84375,
+ "stations": "de Seversky Dock,Stone Market,Wetherill Penal colony,Kushida Port,Tusi Hub,Suydam Orbital,Boscovich Vision,De Lay Orbital,Theodor Winnecke Base,Houtman City,Hansen Refinery"
+ },
+ {
+ "name": "Yupiniang",
+ "pos_x": 68.90625,
+ "pos_y": -36,
+ "pos_z": 107.375,
+ "stations": "Kroehl Platform,Jacobi Vision,Stokes Dock"
+ },
+ {
+ "name": "Yuqui",
+ "pos_x": 42.65625,
+ "pos_y": 42.40625,
+ "pos_z": 57.375,
+ "stations": "Fremont Port,Chang-Diaz Lab,Zelazny Barracks"
+ },
+ {
+ "name": "Yuqui Bab",
+ "pos_x": -59.03125,
+ "pos_y": 96.28125,
+ "pos_z": 13.09375,
+ "stations": "Zajdel Platform,Van Scyoc Terminal"
+ },
+ {
+ "name": "Yuquiyala",
+ "pos_x": 23.46875,
+ "pos_y": -214.125,
+ "pos_z": 67.53125,
+ "stations": "Reeves Mine,Roche Point"
+ },
+ {
+ "name": "Yuracani",
+ "pos_x": -74.9375,
+ "pos_y": -40.25,
+ "pos_z": -169.03125,
+ "stations": "Lanier Terminal,Palmer Landing,Rey's Folly,Ross Enterprise,Maddox Station"
+ },
+ {
+ "name": "Yuragala",
+ "pos_x": 33.71875,
+ "pos_y": 97.53125,
+ "pos_z": -99.78125,
+ "stations": "Gibson Platform,Onufrienko Dock,McMahon Beacon"
+ },
+ {
+ "name": "Yuravandji",
+ "pos_x": 84.84375,
+ "pos_y": 59.65625,
+ "pos_z": -25.09375,
+ "stations": "Wyeth Refinery,Lazutkin Refinery"
+ },
+ {
+ "name": "Yurok",
+ "pos_x": -50.0625,
+ "pos_y": 1.3125,
+ "pos_z": 141.875,
+ "stations": "Janifer Dock,Reynolds Station,Asaro Dock,Gordon Keep,Nicollet Escape"
+ },
+ {
+ "name": "Yurong",
+ "pos_x": -91.46875,
+ "pos_y": -123.5,
+ "pos_z": -39.21875,
+ "stations": "Milnor's Progress"
+ },
+ {
+ "name": "Yuror",
+ "pos_x": -53.875,
+ "pos_y": -111.375,
+ "pos_z": 76.90625,
+ "stations": "Yanai City"
+ },
+ {
+ "name": "Yuruachine",
+ "pos_x": 82.1875,
+ "pos_y": 54.28125,
+ "pos_z": -99.0625,
+ "stations": "Williams Relay,Cardano Base,Klink's Folly"
+ },
+ {
+ "name": "Yurui Wang",
+ "pos_x": 127.59375,
+ "pos_y": -174.375,
+ "pos_z": 107.0625,
+ "stations": "McCool Vision"
+ },
+ {
+ "name": "Yurundhi",
+ "pos_x": 141.625,
+ "pos_y": -84.875,
+ "pos_z": -12.375,
+ "stations": "du Fresne Dock,Eisenstein Ring,Dias Dock"
+ },
+ {
+ "name": "Yuruti",
+ "pos_x": 0.5625,
+ "pos_y": 82.625,
+ "pos_z": -149.75,
+ "stations": "Walker Retreat"
+ },
+ {
+ "name": "Yutula",
+ "pos_x": 77.3125,
+ "pos_y": -115.46875,
+ "pos_z": 145.625,
+ "stations": "Mayer Settlement"
+ },
+ {
+ "name": "Yutumukuni",
+ "pos_x": 57.0625,
+ "pos_y": 16.3125,
+ "pos_z": 136.0625,
+ "stations": "Thomas Vista,Frick Dock"
+ },
+ {
+ "name": "YY Leonis Minoris",
+ "pos_x": 14.3125,
+ "pos_y": 103.46875,
+ "pos_z": -42.1875,
+ "stations": "Hui Vision,Fearn Station,Beaufoy Dock"
+ },
+ {
+ "name": "YZ Canis Minoris",
+ "pos_x": 11.125,
+ "pos_y": 4.6875,
+ "pos_z": -15.21875,
+ "stations": "Ray Freeport"
+ },
+ {
+ "name": "YZ Ceti",
+ "pos_x": -1.1875,
+ "pos_y": -11.8125,
+ "pos_z": -2.15625,
+ "stations": "Clement Orbital,Fuglesang Port,Malchiodi Refinery,Tepper Penal colony"
+ },
+ {
+ "name": "YZ Fornacis",
+ "pos_x": 19.96875,
+ "pos_y": -63.875,
+ "pos_z": -10,
+ "stations": "Kotzebue Gateway,Fanning Beacon,Stasheff Terminal,Lubbock Base,Piccard Ring,Davidson Gateway,Barr Dock,Gentle Orbital,Bova Ring,Dias Observatory,Apt Installation,Charnas Depot"
+ },
+ {
+ "name": "Zac Cechaqu",
+ "pos_x": 110.25,
+ "pos_y": 0.34375,
+ "pos_z": 40.0625,
+ "stations": "Mandel Orbital"
+ },
+ {
+ "name": "Zac Chehoga",
+ "pos_x": 128.34375,
+ "pos_y": -20.625,
+ "pos_z": 8.5625,
+ "stations": "Hencke Base,Fontenay Point"
+ },
+ {
+ "name": "Zac Matlan",
+ "pos_x": 122.46875,
+ "pos_y": -234,
+ "pos_z": 51.75,
+ "stations": "Elst's Inheritance"
+ },
+ {
+ "name": "Zac Og",
+ "pos_x": 169.78125,
+ "pos_y": -113.59375,
+ "pos_z": 93.9375,
+ "stations": "Taylor Jr. Orbital"
+ },
+ {
+ "name": "Zac Uaye",
+ "pos_x": 18,
+ "pos_y": -20.78125,
+ "pos_z": 122.9375,
+ "stations": "Hayashi Platform,Kimura Works,Leiber Hub"
+ },
+ {
+ "name": "Zach",
+ "pos_x": -10.09375,
+ "pos_y": 19.0625,
+ "pos_z": -69.71875,
+ "stations": "Stanley Orbital,Milnor Enterprise,Chiang's Progress"
+ },
+ {
+ "name": "Zacha",
+ "pos_x": 139.75,
+ "pos_y": -206.65625,
+ "pos_z": 26.40625,
+ "stations": "Aller Mines,Mitchell Survey,Mandel Point"
+ },
+ {
+ "name": "Zachigg",
+ "pos_x": 154.75,
+ "pos_y": -87.6875,
+ "pos_z": 16,
+ "stations": "Ritchey Stop"
+ },
+ {
+ "name": "Zagombo",
+ "pos_x": 139.53125,
+ "pos_y": -178.0625,
+ "pos_z": 141.125,
+ "stations": "Haack Orbital,Adams Platform,Frobenius Depot"
+ },
+ {
+ "name": "Zagor",
+ "pos_x": 11.4375,
+ "pos_y": -210.28125,
+ "pos_z": 70.65625,
+ "stations": "Lyne Colony,Mayer Dock,Glushko Landing,Joy Arsenal"
+ },
+ {
+ "name": "Zagorites",
+ "pos_x": 69.96875,
+ "pos_y": -39.03125,
+ "pos_z": -68.65625,
+ "stations": "Leopold Hub"
+ },
+ {
+ "name": "Zagoro",
+ "pos_x": -32.5,
+ "pos_y": -174.59375,
+ "pos_z": -48.75,
+ "stations": "Axon Station,Due Terminal,Denning Terminal,Isaev Dock"
+ },
+ {
+ "name": "Zagotock",
+ "pos_x": 74.125,
+ "pos_y": 96.09375,
+ "pos_z": -56.65625,
+ "stations": "Higginbotham Gateway,Xiaoguan Mines,McKay Orbital,Lawrence Forum,Saunders' Folly"
+ },
+ {
+ "name": "Zamyruaymir",
+ "pos_x": -40.4375,
+ "pos_y": -54.6875,
+ "pos_z": 142.8125,
+ "stations": "Ivins Works,Smith Settlement,Wafer Prospect"
+ },
+ {
+ "name": "Zandu",
+ "pos_x": 37.25,
+ "pos_y": 6.6875,
+ "pos_z": -72.15625,
+ "stations": "Vaucanson Hub,Frimout Horizons,White Depot,Beaufoy Beacon"
+ },
+ {
+ "name": "Zang O",
+ "pos_x": 118.875,
+ "pos_y": -9,
+ "pos_z": -74.3125,
+ "stations": "Koontz Beacon,Zahn Beacon"
+ },
+ {
+ "name": "Zang Tangma",
+ "pos_x": 133.6875,
+ "pos_y": 16.9375,
+ "pos_z": 84.625,
+ "stations": "Johnson Terminal,Pogue Colony,Eanes Enterprise,Isherwood Gateway"
+ },
+ {
+ "name": "Zangpo",
+ "pos_x": -0.875,
+ "pos_y": -177.6875,
+ "pos_z": 80.625,
+ "stations": "McKie Terminal"
+ },
+ {
+ "name": "Zao Gongen",
+ "pos_x": 69.1875,
+ "pos_y": -121.53125,
+ "pos_z": -69.8125,
+ "stations": "Stromgren Hub,Jewitt Survey,Ehrenfried Kegel's Folly"
+ },
+ {
+ "name": "Zao Hi",
+ "pos_x": -98.65625,
+ "pos_y": -84.3125,
+ "pos_z": -115.3125,
+ "stations": "McMonagle Stop"
+ },
+ {
+ "name": "Zao Jiansei",
+ "pos_x": -80.65625,
+ "pos_y": -119.53125,
+ "pos_z": 63.59375,
+ "stations": "Park Dock,Pinzon Colony,Rothfuss Dock,Tenn Horizons"
+ },
+ {
+ "name": "Zaonce",
+ "pos_x": 80.75,
+ "pos_y": 48.75,
+ "pos_z": 69.25,
+ "stations": "Csoma Lab,Ridley Scott,Conway Silo,Sawyer Station,Cixin Installation"
+ },
+ {
+ "name": "Zapakhere",
+ "pos_x": 48.03125,
+ "pos_y": 73.28125,
+ "pos_z": -68.21875,
+ "stations": "Garn Vision,Hernandez Station,Clark Platform,Coelho Keep"
+ },
+ {
+ "name": "Zapalang",
+ "pos_x": 91.0625,
+ "pos_y": -209.5,
+ "pos_z": 9.625,
+ "stations": "McNaught Colony,Ashbrook Station,Lunney Keep"
+ },
+ {
+ "name": "Zaparo",
+ "pos_x": 122.84375,
+ "pos_y": -246.40625,
+ "pos_z": -16.8125,
+ "stations": "Korolev Vision,Seitter Vision,Arfstrom Terminal,Wegner Bastion,Almagro Settlement"
+ },
+ {
+ "name": "Zaporisni",
+ "pos_x": -68.0625,
+ "pos_y": -111.75,
+ "pos_z": -6.71875,
+ "stations": "Riccioli Gateway,Parker Port,Humphreys Depot,Henry Hub"
+ },
+ {
+ "name": "Zara",
+ "pos_x": -12.34375,
+ "pos_y": -121.46875,
+ "pos_z": 83.375,
+ "stations": "Wachmann Settlement,Behnisch Enterprise,Engle Mines"
+ },
+ {
+ "name": "Zaragas",
+ "pos_x": -34.875,
+ "pos_y": -6.0625,
+ "pos_z": 81.96875,
+ "stations": "Jenner Hub,Morin Hub"
+ },
+ {
+ "name": "Zaraluvul",
+ "pos_x": -4.875,
+ "pos_y": 64.21875,
+ "pos_z": -0.8125,
+ "stations": "Plexico Ring,King Orbital"
+ },
+ {
+ "name": "Zaramo",
+ "pos_x": -41.875,
+ "pos_y": -91.75,
+ "pos_z": 6.8125,
+ "stations": "Selberg Installation,Reed City,Scott Holdings"
+ },
+ {
+ "name": "Zaras",
+ "pos_x": 85.96875,
+ "pos_y": 127.4375,
+ "pos_z": 4.75,
+ "stations": "Readdy Terminal"
+ },
+ {
+ "name": "Zarece",
+ "pos_x": 96.09375,
+ "pos_y": 65.71875,
+ "pos_z": 69.1875,
+ "stations": "Bartoe Station,Bose Dock,Brongniart Station,Chretien Terminal,Wescott Station,Clervoy Hub,Gamow Terminal,Atiyah Survey"
+ },
+ {
+ "name": "Zary Orany",
+ "pos_x": -120.65625,
+ "pos_y": -15.1875,
+ "pos_z": -1.625,
+ "stations": "Nemere Vista,Budarin City,Lovelace Orbital,Rutherford Ring,Samuda Holdings,Hudson Refinery"
+ },
+ {
+ "name": "Zarya Manas",
+ "pos_x": -169.25,
+ "pos_y": -96.21875,
+ "pos_z": 27.375,
+ "stations": "Antonio de Andrade Hub,Gelfand Orbital,Bunnell Hub,Baker Prospect,Escobar Bastion"
+ },
+ {
+ "name": "Zavijah",
+ "pos_x": 17.375,
+ "pos_y": 31.09375,
+ "pos_z": 0.5625,
+ "stations": "Junlong Enterprise,Wheelock Ring,Brand Base,Rescue Ship - Wheelock Ring"
+ },
+ {
+ "name": "Zeaex",
+ "pos_x": 27.53125,
+ "pos_y": 0.8125,
+ "pos_z": 26.84375,
+ "stations": "Wheelock Settlement,Karl Diesel City,Tryggvason Orbital,Precourt Enterprise,Moffitt Town"
+ },
+ {
+ "name": "Zearla",
+ "pos_x": 26.15625,
+ "pos_y": -52.9375,
+ "pos_z": 31.625,
+ "stations": "Payson Hub,Perga Port,Chomsky Camp,Bulgakov Base,Qian Depot"
+ },
+ {
+ "name": "Zeessze",
+ "pos_x": -19.8125,
+ "pos_y": -3.25,
+ "pos_z": -14.28125,
+ "stations": "Rosseland Gateway,Nicollier Hangar,Perry Vision,Hoyle Fort,Cauchy Gateway"
+ },
+ {
+ "name": "Zelacanto",
+ "pos_x": 66.40625,
+ "pos_y": -129.5625,
+ "pos_z": 6.15625,
+ "stations": "Peltier Vision,Druillet Dock,Tousey Colony,Robinson Installation,Phillifent Vista"
+ },
+ {
+ "name": "Zelada",
+ "pos_x": -58.875,
+ "pos_y": -21.53125,
+ "pos_z": -54.84375,
+ "stations": "Lanier Colony,Gotlieb Relay,Hearthrug Gruvara,Port Zelada"
+ },
+ {
+ "name": "Zelano",
+ "pos_x": 27.78125,
+ "pos_y": -83,
+ "pos_z": 16.6875,
+ "stations": "Kowal City,Shavyrin Terminal,Aitken Terminal,Mueller Station,Fan Vision,Melotte Hub,Riccioli Station,Gurshtein City,Naburimannu Gateway,Asprin Survey,Hornoch Beacon,Laumer Holdings"
+ },
+ {
+ "name": "Zelao",
+ "pos_x": 136.5,
+ "pos_y": -181.34375,
+ "pos_z": 56.8125,
+ "stations": "Stiles Landing,Reid Point"
+ },
+ {
+ "name": "Zelarna",
+ "pos_x": 133.125,
+ "pos_y": 43.0625,
+ "pos_z": -23.75,
+ "stations": "Searfoss Outpost"
+ },
+ {
+ "name": "Zememede",
+ "pos_x": 91.5625,
+ "pos_y": 15.84375,
+ "pos_z": -140.71875,
+ "stations": "Elwood Depot"
+ },
+ {
+ "name": "Zemete",
+ "pos_x": -12.71875,
+ "pos_y": -92,
+ "pos_z": 131.28125,
+ "stations": "Hoffmeister Works"
+ },
+ {
+ "name": "Zemetii",
+ "pos_x": 68.125,
+ "pos_y": -140.375,
+ "pos_z": -51.59375,
+ "stations": "Argelander Port,Arrhenius Orbital,Rich Port,Gurney Orbital,Herschel Orbital,Chertovsky Vision,Killough Works,Sitter Station,Ray Bastion,Yuzhe Dock,Galle Orbital,Matheson Refinery,Kirby Prospect,Karlsefni Refinery"
+ },
+ {
+ "name": "Zemez",
+ "pos_x": -11.6875,
+ "pos_y": 9.5625,
+ "pos_z": 60.3125,
+ "stations": "Crown Dock"
+ },
+ {
+ "name": "Zemlia",
+ "pos_x": -182.96875,
+ "pos_y": -35.21875,
+ "pos_z": -63.125,
+ "stations": "Berezin Point,Janszoon's Inheritance"
+ },
+ {
+ "name": "Zemliang",
+ "pos_x": -37.59375,
+ "pos_y": -24.65625,
+ "pos_z": 127.1875,
+ "stations": "Jordan's Progress,Allen Base,Kuhn Works"
+ },
+ {
+ "name": "Zemlya",
+ "pos_x": 51.90625,
+ "pos_y": 30.28125,
+ "pos_z": 158.5,
+ "stations": "Burkin Works,Schade Oasis"
+ },
+ {
+ "name": "Zemlyaqa",
+ "pos_x": 61.46875,
+ "pos_y": -57.40625,
+ "pos_z": -40.96875,
+ "stations": "Madsen Point"
+ },
+ {
+ "name": "Zende",
+ "pos_x": 226.0625,
+ "pos_y": -134.3125,
+ "pos_z": -1.75,
+ "stations": "Vavrova Landing,Vuia Survey,Ford Colony,Brorsen Prospect"
+ },
+ {
+ "name": "Zenmait",
+ "pos_x": 55.21875,
+ "pos_y": -182.5,
+ "pos_z": 60.4375,
+ "stations": "Michael Colony"
+ },
+ {
+ "name": "Zenmaku",
+ "pos_x": -90.46875,
+ "pos_y": 19.375,
+ "pos_z": -62.71875,
+ "stations": "Lucretius Survey"
+ },
+ {
+ "name": "Zenufangwe",
+ "pos_x": 78.15625,
+ "pos_y": 94.34375,
+ "pos_z": -42.125,
+ "stations": "Murray Port,Longyear Survey,Edwards Hub"
+ },
+ {
+ "name": "Zenus",
+ "pos_x": -70.4375,
+ "pos_y": -97.21875,
+ "pos_z": -11.5,
+ "stations": "Gunn Station,Alexander Port,Abbott Beacon,Walker Observatory,Landis Installation"
+ },
+ {
+ "name": "Zenusheper",
+ "pos_x": -116.75,
+ "pos_y": 117.0625,
+ "pos_z": -57.3125,
+ "stations": "Taine Depot"
+ },
+ {
+ "name": "Zenut",
+ "pos_x": 123.625,
+ "pos_y": 36.6875,
+ "pos_z": 102.78125,
+ "stations": "Knight Mine,Treshchov Mines,Gann Installation"
+ },
+ {
+ "name": "Zenutet",
+ "pos_x": 55.90625,
+ "pos_y": -72.90625,
+ "pos_z": 85.9375,
+ "stations": "Naubakht Platform,Hussey Hub,Bartolomeu de Gusmao Ring"
+ },
+ {
+ "name": "Zephyrus",
+ "pos_x": -21.84375,
+ "pos_y": 12.59375,
+ "pos_z": -60.75,
+ "stations": "EY06,Poincare City,King Depot"
+ },
+ {
+ "name": "Zeta Aquilae",
+ "pos_x": -60.84375,
+ "pos_y": 4.03125,
+ "pos_z": 56.34375,
+ "stations": "Oefelein Plant,Yolen Depot,Mohmand Holdings"
+ },
+ {
+ "name": "Zeta Fornacis",
+ "pos_x": 30.8125,
+ "pos_y": -92.8125,
+ "pos_z": -41.875,
+ "stations": "Uto Terminal,Webb Terminal,Wales Hub,Addams Port,Ptack Vision,Gloss Gateway,Kidinnu Hub,Oosterhoff Dock,Cowell Hub,Wendelin Station"
+ },
+ {
+ "name": "Zeta Horologii",
+ "pos_x": 89.125,
+ "pos_y": -132.90625,
+ "pos_z": 6.03125,
+ "stations": "May Port,Patry Gateway,August von Steinheil Barracks,Goldschmidt Horizons"
+ },
+ {
+ "name": "Zeta Leporis",
+ "pos_x": 42.125,
+ "pos_y": -24.5,
+ "pos_z": -50.9375,
+ "stations": "McMahon Silo,O'Connor Enterprise,Stephenson Beacon,Borlaug Survey"
+ },
+ {
+ "name": "Zeta Lupi",
+ "pos_x": 68.5,
+ "pos_y": 9.25,
+ "pos_z": 94.78125,
+ "stations": "Norman Platform,Keyes Port,Hatch's Memorial"
+ },
+ {
+ "name": "Zeta Microscopii",
+ "pos_x": -6.53125,
+ "pos_y": -77.3125,
+ "pos_z": 85.375,
+ "stations": "Noli Station,Huberath Station,Eilenberg Enterprise,Warner Hub,Gardner Port,Andersson Reach,Sagan Gateway,Davidson Port,Cousteau Station,Barcelo Ring,Zebrowski Landing,Bailey Horizons,Gulyaev Reach,Schaumasse Barracks,Snyder's Claim,Stafford Vision"
+ },
+ {
+ "name": "Zeta Pictoris",
+ "pos_x": 93.375,
+ "pos_y": -66.46875,
+ "pos_z": -20.875,
+ "stations": "Hickman Orbital,Fu Point"
+ },
+ {
+ "name": "Zeta Telescopii",
+ "pos_x": 28.9375,
+ "pos_y": -37.4375,
+ "pos_z": 117,
+ "stations": "Shawl Station,Dyomin Installation,Aldiss' Folly,Altshuller Station,Greenleaf Base"
+ },
+ {
+ "name": "Zeta Trianguli Australis",
+ "pos_x": 24.65625,
+ "pos_y": -10.25,
+ "pos_z": 29.125,
+ "stations": "Guest City,Brackett Prospect,Ampere Hub,Ramsbottom Port,Hartlib Barracks"
+ },
+ {
+ "name": "Zeta Tucanae",
+ "pos_x": 13.5,
+ "pos_y": -22.15625,
+ "pos_z": 10.53125,
+ "stations": "Velidhu Dream,Elswick,Noon Survey"
+ },
+ {
+ "name": "Zeta Volantis",
+ "pos_x": 126.25,
+ "pos_y": -53.40625,
+ "pos_z": 33.03125,
+ "stations": "Darwin Enterprise,Nicholson Prospect,Clayton Survey"
+ },
+ {
+ "name": "Zeta-1 Reticuli",
+ "pos_x": 26.25,
+ "pos_y": -28.78125,
+ "pos_z": 4.0625,
+ "stations": "Brunel Colony"
+ },
+ {
+ "name": "Zeus",
+ "pos_x": 21.4375,
+ "pos_y": -52.8125,
+ "pos_z": -25.71875,
+ "stations": "KatherineB,Hillier 1,Narita Enterprise,The Midas"
+ },
+ {
+ "name": "Zhan Wen",
+ "pos_x": -3.625,
+ "pos_y": -98.59375,
+ "pos_z": 88.4375,
+ "stations": "Yuzhe Gateway,Lundmark Gateway,Morgan Dock,Forbes' Inheritance"
+ },
+ {
+ "name": "Zhana",
+ "pos_x": 40.125,
+ "pos_y": 136.5,
+ "pos_z": 36.84375,
+ "stations": "Popovich Station"
+ },
+ {
+ "name": "Zhang",
+ "pos_x": -84.34375,
+ "pos_y": -119.5,
+ "pos_z": 58.09375,
+ "stations": "McAllaster Relay,Stevens Camp,Oshima Base"
+ },
+ {
+ "name": "Zhang Fei",
+ "pos_x": 120.59375,
+ "pos_y": 3.96875,
+ "pos_z": -34.8125,
+ "stations": "Kovalevsky Point,Serebrov Horizons"
+ },
+ {
+ "name": "Zhang Sheng",
+ "pos_x": 117.28125,
+ "pos_y": -199.6875,
+ "pos_z": -1.46875,
+ "stations": "Harrington City,Parker Dock,Donati Port,Green Enterprise,Beliaev Barracks"
+ },
+ {
+ "name": "Zhang Yu",
+ "pos_x": 183.5625,
+ "pos_y": -115.1875,
+ "pos_z": -4.34375,
+ "stations": "Herschel City,Freas Orbital"
+ },
+ {
+ "name": "Zhang Yun",
+ "pos_x": 36.28125,
+ "pos_y": -62.375,
+ "pos_z": 99.59375,
+ "stations": "Jefferies Horizons,Kazantsev Point,de Seversky Mines"
+ },
+ {
+ "name": "Zhangana",
+ "pos_x": 86.59375,
+ "pos_y": -134.6875,
+ "pos_z": 21.4375,
+ "stations": "Yerka Holdings,Matthews Installation,Holland's Progress"
+ },
+ {
+ "name": "Zhangda",
+ "pos_x": -65.4375,
+ "pos_y": 98.0625,
+ "pos_z": -20.71875,
+ "stations": "Tennyson d'Eyncourt Hangar,Monge Station,Phillifent Enterprise"
+ },
+ {
+ "name": "Zhao",
+ "pos_x": 108.6875,
+ "pos_y": -113.46875,
+ "pos_z": 74.0625,
+ "stations": "Bell Dock,Bolton Orbital,Maupertuis Dock,Weinbaum Legacy,Rucker Depot,Tombaugh Terminal,Webb Settlement,Fairey Hub,Common Landing,Mainzer Landing,Mooz Station"
+ },
+ {
+ "name": "Zhao Gong",
+ "pos_x": 162.46875,
+ "pos_y": -89.875,
+ "pos_z": 73.84375,
+ "stations": "Giraud Mine"
+ },
+ {
+ "name": "Zhao Jin",
+ "pos_x": 125.25,
+ "pos_y": -43.875,
+ "pos_z": 27.53125,
+ "stations": "Evans Installation,Wilson Ring,Elst Hub,MacDonald Lab,Piazzi Ring"
+ },
+ {
+ "name": "Zhao Tzu",
+ "pos_x": -23.90625,
+ "pos_y": -34.28125,
+ "pos_z": -95,
+ "stations": "Salam Dock,Ramsay Refinery,Schrodinger Hub,Chilton Camp"
+ },
+ {
+ "name": "Zhaoxian",
+ "pos_x": -61.90625,
+ "pos_y": -104.40625,
+ "pos_z": 120.96875,
+ "stations": "Hughes Mines"
+ },
+ {
+ "name": "Zhi",
+ "pos_x": 2.1875,
+ "pos_y": -20.53125,
+ "pos_z": -8.96875,
+ "stations": "Metcalf Hub,Lister Hangar,Salk Settlement,Rukavishnikov Station"
+ },
+ {
+ "name": "Zhonawasi",
+ "pos_x": -53.78125,
+ "pos_y": -144.34375,
+ "pos_z": 2.21875,
+ "stations": "Wescott Outpost,Turtledove Point"
+ },
+ {
+ "name": "Zhongwe",
+ "pos_x": 124.9375,
+ "pos_y": -94.71875,
+ "pos_z": -11.875,
+ "stations": "Angstrom Outpost"
+ },
+ {
+ "name": "Zhonvenk",
+ "pos_x": -22.3125,
+ "pos_y": -59.84375,
+ "pos_z": -96,
+ "stations": "Fanning Barracks,MacCurdy Landing,Watt Port"
+ },
+ {
+ "name": "Zhou Maola",
+ "pos_x": 96.8125,
+ "pos_y": 91.3125,
+ "pos_z": -5.0625,
+ "stations": "Euclid Dock,Pasteur Terminal"
+ },
+ {
+ "name": "Zhou Wang",
+ "pos_x": -12.5,
+ "pos_y": -137.34375,
+ "pos_z": 35.1875,
+ "stations": "Rosseland Station,Gustav Sporer City"
+ },
+ {
+ "name": "Zhu Baba",
+ "pos_x": 57.375,
+ "pos_y": -124.9375,
+ "pos_z": 51.53125,
+ "stations": "Nahavandi Port,Goldschmidt Station,Weaver Installation"
+ },
+ {
+ "name": "Zhu Ji",
+ "pos_x": -131.71875,
+ "pos_y": -108.0625,
+ "pos_z": 17.375,
+ "stations": "Curie Enterprise,Svavarsson Depot"
+ },
+ {
+ "name": "Zhu Vedani",
+ "pos_x": 80.09375,
+ "pos_y": -13.78125,
+ "pos_z": -96.25,
+ "stations": "Volterra Hub,Pratchett Vision,Hoyle Hub,Morris Enterprise,Herbert Point"
+ },
+ {
+ "name": "Zhu Veddi",
+ "pos_x": 115.34375,
+ "pos_y": -177.03125,
+ "pos_z": 25.9375,
+ "stations": "Chandra Prospect,Rechtin Port,Qureshi Vision,Shipton Installation"
+ },
+ {
+ "name": "Zhuaha",
+ "pos_x": 160.28125,
+ "pos_y": -166.34375,
+ "pos_z": 101.15625,
+ "stations": "Patry Vision,Abe Settlement,Brooks Enterprise"
+ },
+ {
+ "name": "Zhuala",
+ "pos_x": 0.25,
+ "pos_y": -125.40625,
+ "pos_z": 38.84375,
+ "stations": "Woodroffe Beacon,Merritt Vision,Lunney Orbital,Kidinnu Orbital,Lee Orbital"
+ },
+ {
+ "name": "Zhuang",
+ "pos_x": 22.78125,
+ "pos_y": -196.03125,
+ "pos_z": -14.34375,
+ "stations": "Hague Dock,Wigura Colony,Morris Vision,Kennan Depot"
+ },
+ {
+ "name": "Zhuar",
+ "pos_x": 12.15625,
+ "pos_y": 172.46875,
+ "pos_z": -44.125,
+ "stations": "Readdy Platform,Hopkins Survey"
+ },
+ {
+ "name": "Zhuaruparci",
+ "pos_x": -43.59375,
+ "pos_y": -69.46875,
+ "pos_z": 64.34375,
+ "stations": "Lawhead Base"
+ },
+ {
+ "name": "Zi Goan",
+ "pos_x": 3,
+ "pos_y": -118.4375,
+ "pos_z": 120.78125,
+ "stations": "Kazantsev Installation,Gabrielli Survey,Carpenter Platform"
+ },
+ {
+ "name": "Zi Guo Wu",
+ "pos_x": 5.3125,
+ "pos_y": -218.0625,
+ "pos_z": 61.0625,
+ "stations": "Christy Dock,Charlois Hub,Resnick Plant,Hulse Port,Schmidt's Folly"
+ },
+ {
+ "name": "Zi Yines",
+ "pos_x": -19.65625,
+ "pos_y": -159.46875,
+ "pos_z": -67.84375,
+ "stations": "Hirn Terminal,Noli Camp,Gurshtein Vision,Kratman Base"
+ },
+ {
+ "name": "Zi Yomi",
+ "pos_x": -116,
+ "pos_y": -20.65625,
+ "pos_z": 82.15625,
+ "stations": "Britnev Terminal,Charnas Port,Fox Port,Busch Ring,Arnarson Hub,Wood Enterprise,Lupoff Port,Finney Hub,Hadamard's Claim"
+ },
+ {
+ "name": "Zibal",
+ "pos_x": 14.25,
+ "pos_y": -84.9375,
+ "pos_z": -68.03125,
+ "stations": "Hire Port,Veach Vision"
+ },
+ {
+ "name": "Zlota",
+ "pos_x": 106.1875,
+ "pos_y": -2.6875,
+ "pos_z": 67.375,
+ "stations": "Nusslein-Volhard Settlement,Seega Hub"
+ },
+ {
+ "name": "Zlota Lung",
+ "pos_x": 45.5625,
+ "pos_y": 77.34375,
+ "pos_z": 88.6875,
+ "stations": "Tyson Beacon"
+ },
+ {
+ "name": "Zlotaikua",
+ "pos_x": -11.875,
+ "pos_y": -102,
+ "pos_z": 135.96875,
+ "stations": "Gutierrez Outpost,Nyberg Survey,Ross Works"
+ },
+ {
+ "name": "Zlotrighu",
+ "pos_x": 121.5625,
+ "pos_y": -145.3125,
+ "pos_z": 116,
+ "stations": "Schwarzschild Dock,Clarke Bastion"
+ },
+ {
+ "name": "Zlotrimi",
+ "pos_x": -16,
+ "pos_y": -23.21875,
+ "pos_z": 139.5625,
+ "stations": "Hieb Terminal,Fraley Orbital,Wrangell Port,Arber Orbital,Maudslay Orbital,Gubarev Port,Katzenstein Terminal,Ising Terminal,Nowak Oasis,Ryumin Reach,McCoy Survey"
+ },
+ {
+ "name": "Zmey Goab",
+ "pos_x": -24.0625,
+ "pos_y": -79.96875,
+ "pos_z": 76.78125,
+ "stations": "Eisele Market,Gibson Laboratory,Wells Survey,Smith Enterprise,Nikolayev's Inheritance"
+ },
+ {
+ "name": "Zmeyaaya",
+ "pos_x": -82.21875,
+ "pos_y": -18.5,
+ "pos_z": 108.1875,
+ "stations": "Houtman Base,Wilhelm Dock"
+ },
+ {
+ "name": "Zmeyang",
+ "pos_x": 27.84375,
+ "pos_y": -10.4375,
+ "pos_z": 124.15625,
+ "stations": "Wolf Horizons,Kubasov's Folly,Henry Relay"
+ },
+ {
+ "name": "Zochna",
+ "pos_x": 115.78125,
+ "pos_y": -10.3125,
+ "pos_z": 5.375,
+ "stations": "Khan Terminal,Born Dock"
+ },
+ {
+ "name": "Zoelang",
+ "pos_x": 89.28125,
+ "pos_y": -146.84375,
+ "pos_z": -29.8125,
+ "stations": "Folland Hub,Tietjen Terminal,Gabrielli's Progress,Plaskett Hub"
+ },
+ {
+ "name": "Zoelati",
+ "pos_x": 100.65625,
+ "pos_y": -174.3125,
+ "pos_z": -23.78125,
+ "stations": "McCrea Vision,Finlay-Freundlich Survey,Hinz Base,Antonov Port,Vancouver Prison"
+ },
+ {
+ "name": "Zomba",
+ "pos_x": -104.53125,
+ "pos_y": 0.59375,
+ "pos_z": 2.75,
+ "stations": "Dozois Orbital,Killough Relay,Vesalius Terminal"
+ },
+ {
+ "name": "Zombivaroa",
+ "pos_x": 100.5625,
+ "pos_y": -119.5625,
+ "pos_z": 77.875,
+ "stations": "Vinge Landing,Titius Prospect,Resnik Landing"
+ },
+ {
+ "name": "Zombo",
+ "pos_x": -139.5625,
+ "pos_y": 30.375,
+ "pos_z": -20.90625,
+ "stations": "Caryanda Horizons,Klink Horizons,Chiang Hub"
+ },
+ {
+ "name": "Zoquagsang",
+ "pos_x": 57.90625,
+ "pos_y": 80.15625,
+ "pos_z": 146.09375,
+ "stations": "Bernard City,Bain Hub,Samuda Penal colony"
+ },
+ {
+ "name": "Zoquala",
+ "pos_x": -76.78125,
+ "pos_y": -73.0625,
+ "pos_z": -70.5625,
+ "stations": "Scalzi Hub"
+ },
+ {
+ "name": "Zoqui",
+ "pos_x": 47.375,
+ "pos_y": 59.84375,
+ "pos_z": 76.5625,
+ "stations": "Helmholtz Landing,Glidden Holdings"
+ },
+ {
+ "name": "Zoqui Xuang",
+ "pos_x": -83.15625,
+ "pos_y": -15.15625,
+ "pos_z": 70.46875,
+ "stations": "Hoffman Port,Hoften Enterprise,Wright Dock,Coke Terminal"
+ },
+ {
+ "name": "Zorya",
+ "pos_x": -50.46875,
+ "pos_y": -14.8125,
+ "pos_z": -22.59375,
+ "stations": "Snodgrass Station,Tiptree Port,Taine Enterprise,Cantor Terminal,Steele Hub"
+ },
+ {
+ "name": "Zorya Nong",
+ "pos_x": 18.59375,
+ "pos_y": -104.1875,
+ "pos_z": -90.28125,
+ "stations": "Michalitsianos Port,Struzan Enterprise,Fourier Enterprise,Qushji Terminal,Weber Bastion"
+ },
+ {
+ "name": "Zorya Wujis",
+ "pos_x": -72.5625,
+ "pos_y": -51,
+ "pos_z": 53.59375,
+ "stations": "Abel Enterprise,Chebyshev Terminal"
+ },
+ {
+ "name": "Zoryacenuo",
+ "pos_x": 121.96875,
+ "pos_y": -59.71875,
+ "pos_z": 28.78125,
+ "stations": "Shaw Terminal"
+ },
+ {
+ "name": "Zoryaco",
+ "pos_x": -32.9375,
+ "pos_y": -125.96875,
+ "pos_z": 80.65625,
+ "stations": "Fontenay City"
+ },
+ {
+ "name": "Zoryak",
+ "pos_x": 133.6875,
+ "pos_y": -109.84375,
+ "pos_z": -62.78125,
+ "stations": "Allen St. John Colony,Krigstein Port,Alten's Folly"
+ },
+ {
+ "name": "Zoryampt",
+ "pos_x": 22.21875,
+ "pos_y": 35.8125,
+ "pos_z": 131,
+ "stations": "Musabayev Landing,Fossum Platform"
+ },
+ {
+ "name": "Zoryang",
+ "pos_x": -59.21875,
+ "pos_y": -87.125,
+ "pos_z": -33.21875,
+ "stations": "Forrester Stop"
+ },
+ {
+ "name": "Zorynhili",
+ "pos_x": -30.75,
+ "pos_y": 99.8125,
+ "pos_z": 83.8125,
+ "stations": "Wiberg Hub,Macgregor Silo,Bain Vision"
+ },
+ {
+ "name": "Zorynian",
+ "pos_x": 146.21875,
+ "pos_y": -114.4375,
+ "pos_z": 85.25,
+ "stations": "Tupolev Works,Alexandria Silo"
+ },
+ {
+ "name": "Zorynici",
+ "pos_x": -17.96875,
+ "pos_y": 91.96875,
+ "pos_z": 104.46875,
+ "stations": "Carson's Claim"
+ },
+ {
+ "name": "Zosi",
+ "pos_x": -20.03125,
+ "pos_y": 96.9375,
+ "pos_z": -37.625,
+ "stations": "Citi Enterprise,Vizcaino Port,Flinders Enterprise,Gibbs Depot,Whitelaw Vision"
+ },
+ {
+ "name": "Zosia",
+ "pos_x": -16.75,
+ "pos_y": 19.59375,
+ "pos_z": -60.0625,
+ "stations": "Diamond Base,Sharp Enterprise,Beatty Observatory"
+ },
+ {
+ "name": "Zosian Tse",
+ "pos_x": 20,
+ "pos_y": -159.21875,
+ "pos_z": -30.625,
+ "stations": "Cellarius Ring,Connes Ring,Dunlop Port,Gehry Penal colony"
+ },
+ {
+ "name": "Zosinambure",
+ "pos_x": 92.71875,
+ "pos_y": -23.9375,
+ "pos_z": -90.375,
+ "stations": "Hiroyuki Plant,Tenn Dock,Schade's Claim"
+ },
+ {
+ "name": "Zuben Elgenubi",
+ "pos_x": 19.6875,
+ "pos_y": 46.09375,
+ "pos_z": 56.84375,
+ "stations": "Sternberg Terminal"
+ },
+ {
+ "name": "Zugen",
+ "pos_x": -23.375,
+ "pos_y": 5.40625,
+ "pos_z": 38.40625,
+ "stations": "Soddy Hangar"
+ },
+ {
+ "name": "Zuruaha",
+ "pos_x": 137.28125,
+ "pos_y": 53.1875,
+ "pos_z": -11.8125,
+ "stations": "O'Connor Dock,White Terminal,Palmer Prospect,Savitskaya Settlement"
+ },
+ {
+ "name": "Zurukuruni",
+ "pos_x": -117.15625,
+ "pos_y": -31.46875,
+ "pos_z": 97.9375,
+ "stations": "Wolfe Settlement,Dall Platform,Godel Camp,Lehtonen Base"
+ },
+ {
+ "name": "Zurum",
+ "pos_x": -42.75,
+ "pos_y": -115.1875,
+ "pos_z": 120.65625,
+ "stations": "Alexander Ring"
+ },
+ {
+ "name": "Zvaithhogg",
+ "pos_x": 81.0625,
+ "pos_y": -129.3125,
+ "pos_z": -3.5,
+ "stations": "Laming Vision,Bierce Depot,Blaauw Prospect,Hogg Port,Karachkina Orbital"
+ },
+ {
+ "name": "Zvaiti",
+ "pos_x": -43.21875,
+ "pos_y": -66.6875,
+ "pos_z": -114.25,
+ "stations": "Hume Retreat,Langsdorff Mine"
+ },
+ {
+ "name": "Zvaizdikis",
+ "pos_x": 44.65625,
+ "pos_y": 35.0625,
+ "pos_z": -104.625,
+ "stations": "Buckland Platform,Zewail Beacon"
+ },
+ {
+ "name": "Zvaizgan",
+ "pos_x": 53.5,
+ "pos_y": -20.875,
+ "pos_z": 60.375,
+ "stations": "Ellis Hub"
+ },
+ {
+ "name": "Zvorsingin",
+ "pos_x": -135.0625,
+ "pos_y": -22.15625,
+ "pos_z": 29.90625,
+ "stations": "Bykovsky Base"
+ },
+ {
+ "name": "Zvoruna",
+ "pos_x": 129.09375,
+ "pos_y": 22.25,
+ "pos_z": 25.21875,
+ "stations": "Debye Market,Swift Installation,Gardner Enterprise,Belyayev Gateway,Maine Survey,Effinger Depot"
+ },
+ {
+ "name": "ZZ Piscium",
+ "pos_x": -27.65625,
+ "pos_y": -35.34375,
+ "pos_z": 0.875,
+ "stations": "Buffett Survey,Adamson Arsenal,Wilcutt Lab"
+ }
+]
diff --git a/data/milkyway-ed.json b/data/milkyway-ed.json
new file mode 100644
index 0000000..26b35f6
--- /dev/null
+++ b/data/milkyway-ed.json
@@ -0,0 +1,272 @@
+{
+
+ "quadrants" : {
+ "The Orion Spur" : {
+ "x":0, "z":0, "rotate":0
+ },
+ "Galactic Core Region" : {
+ "x":974, "z":27333, "rotate":0
+ },
+ "1st quadrant" : {
+ "x":-15000, "z":5000, "rotate":0
+ },
+ "4th quadrant" : {
+ "x":15000, "z":5000, "rotate":0
+ },
+ "2nd quadrant" : {
+ "x":-10000, "z":-2000, "rotate":0
+ },
+ "3rd quadrant" : {
+ "x":10000, "z":-2000, "rotate":0
+ }
+ },
+
+ "arms" : {
+ "The Orion Spur" : [
+ {"x":-16284, "z":16823, "rotate":90},
+ {"x":-7265, "z":4226, "rotate":-35},
+ {"x":6858, "z":-576, "rotate":0}
+ ],
+ "The outer Arm vacuus" : [
+ {"x":10, "z":-14500, "rotate":0}
+ ],
+ "The Sagittarius Arm" : [
+ {"x":5379, "z":4768, "rotate":0}
+ ],
+ "The Carina-Sagittarius Arm" : [
+ {"x":22458, "z":10237, "rotate":45},
+ {"x":29632, "z":25285, "rotate":82},
+ {"x":28962, "z":40634, "rotate":105},
+ {"x":17446, "z":58185, "rotate":-40}
+ ],
+ "The new outer Arm" : [
+ {"x":-31158, "z":16529, "rotate":90},
+ {"x":-28281, "z":3070, "rotate":112},
+ {"x":-18972, "z":-8546, "rotate":-35}
+
+ ],
+ "The Perseus Arm" : [
+ {"x":-19961, "z":30870, "rotate":60 },
+ {"x":-22778, "z":10633, "rotate":110 },
+ {"x":-7525, "z":-3597, "rotate":-25 },
+ {"x":8039, "z":-7019, "rotate":0 },
+ {"x":32905, "z":4565, "rotate":45 }
+ ],
+ "The Norma Arm" : [
+ {"x":7944, "z":17960, "rotate":40 },
+ {"x":13524, "z":29918, "rotate":90 },
+ {"x":7498, "z":40348, "rotate":-35 }
+ ],
+ "The Cygnus Arm" : [
+ {"x":-7724, "z":43523, "rotate":0 },
+ {"x":-22353, "z":37643, "rotate":38 }
+ ],
+ "The Scutum-Centaurus Arm" : [
+ {"x":-33364, "z":46016, "rotate":45 },
+ {"x":-15742, "z":54759, "rotate":0 },
+ {"x":-334, "z":53331, "rotate":-14 },
+ {"x":14300, "z":46140, "rotate":-38 },
+ {"x":19815, "z":31418, "rotate":90 },
+ {"x":16637, "z":17458, "rotate":48 }
+ ],
+ "The near 3 KPC Arm" : [
+ {"x":5765, "z":22639, "rotate":45 }
+ ],
+ "The far 3 KPC Arm" : [
+ {"x":-4906 ,"z":28535, "rotate":45 }
+ ]
+ },
+
+ "gaps" : {
+
+ },
+
+ "others" : {
+ "Sagittarius A*" : [
+ {"x":25 , "z":25900, "rotate":0}
+ ],
+
+
+
+"The Abyss" : [
+ {"x":-8420, "z":63240, "rotate":0}
+ ],
+"The Abyssal Plain" : [
+ {"x":-21660 , "z":55680, "rotate":0}
+ ],
+"Angustia" : [
+ {"x":-26110 , "z":6960, "rotate":0}
+ ],
+"The Anthor Patch" : [
+ {"x":-14900 , "z":23310, "rotate":0}
+ ],
+"The Ascendance" : [
+ {"x":18960 , "z":32800, "rotate":0}
+ ],
+"The Bleak Lands" : [
+ {"x":9360 , "z":47260, "rotate":0}
+ ],
+"Boreas" : [
+ {"x":-5780 , "z":43240, "rotate":0}
+ ],
+"Centaurus Reach" : [
+ {"x":21420 , "z":11740, "rotate":0}
+ ],
+"Circinus Transit" : [
+ {"x":13760 , "z":16540, "rotate":0}
+ ],
+"Colonia" : [
+ {"x":-9560 , "z":19950, "rotate":0}
+ ],
+"The Dryman Ridge" : [
+ {"x":22720 , "z":20010, "rotate":0}
+ ],
+"Eastern Neutron Fields" : [
+ {"x":2680 , "z":24780, "rotate":0}
+ ],
+"Eurus" : [
+ {"x":10880 , "z":36760, "rotate":0}
+ ],
+"The Fallows" : [
+ {"x":-19040 , "z":13200, "rotate":0}
+ ],
+"The Festival Grounds" : [
+ {"x":-10870 , "z":17650, "rotate":0}
+ ],
+"The Formidine Rift" : [
+ {"x":-14220 , "z":-5280, "rotate":0}
+ ],
+"Galactic Aphelion" : [
+ {"x":-2320 , "z":56180, "rotate":0}
+ ],
+"The Gallipolis" : [
+ {"x":6560 , "z":20070, "rotate":0}
+ ],
+"Hawking's Gap" : [
+ {"x":16360 , "z":12820, "rotate":0}
+ ],
+"Hipparcos Basin" : [
+ {"x":22960 , "z":34280, "rotate":0}
+ ],
+"Hyponia" : [
+ {"x":-22220 , "z":24780, "rotate":0}
+ ],
+"Ishtar" : [
+ {"x":-21335 , "z":39365, "rotate":0}
+ ],
+"Lin-Shu Hollow" : [
+ {"x":6870 , "z":7335, "rotate":0}
+ ],
+"Mare Desperationis" : [
+ {"x":-22240 , "z":42680, "rotate":0}
+ ],
+"Masefield's Ocean" : [
+ {"x":-2080 , "z":55045, "rotate":0}
+ ],
+"The Norma Expanse" : [
+ {"x":5320 , "z":14900, "rotate":0}
+ ],
+"Nyauthai Ripple" : [
+ {"x":-7465 , "z":55245, "rotate":0}
+ ],
+"Orio-Perseus Conflux" : [
+ {"x":-15400 , "z":32680, "rotate":0}
+ ],
+"The Orion Complex" : [
+ {"x":730 , "z":-1145, "rotate":0}
+ ],
+
+
+
+"Orio-Perseus Gap" : [
+ {"x":-4160 , "z":-960, "rotate":0}
+ ],
+"The Orion Spur" : [
+ {"x":2180 , "z":800, "rotate":0}
+ ],
+"The Orion Spur Shallows" : [
+ {"x":17740 , "z":2570, "rotate":0}
+ ],
+"The Outer Arm Rift" : [
+ {"x":-29650 , "z":28640, "rotate":0}
+ ],
+"The Outer Arm Vacuus" : [
+ {"x":2020 , "z":-12820, "rotate":0}
+ ],
+"The Perseus Crags" : [
+ {"x":-22130 , "z":12220, "rotate":0}
+ ],
+"The Perseus Fade" : [
+ {"x":22140 , "z":-5520, "rotate":0}
+ ],
+"The Perseus Transit" : [
+ {"x":11400 , "z":-3620, "rotate":0}
+ ],
+"Perseus Stem" : [
+ {"x":730 , "z":36190, "rotate":0}
+ ],
+"Sagittarius Gap" : [
+ {"x":1470 , "z":3010, "rotate":0}
+ ],
+"The Scar" : [
+ {"x":-22830 , "z":26005, "rotate":0}
+ ],
+"Scutum-Sagittarii Conflux" : [
+ {"x":-3280 , "z":9620, "rotate":0}
+ ],
+"Silentium" : [
+ {"x":18480 , "z":57400, "rotate":0}
+ ],
+
+
+
+
+"The Sidereal Wall" : [
+ {"x":-250 , "z":15140, "rotate":0}
+ ],
+"The Solitude Void" : [
+ {"x":3660 , "z":63600, "rotate":0}
+ ],
+"Styx" : [
+ {"x":1430 , "z":45370, "rotate":0}
+ ],
+"Tenebris" : [
+ {"x":33620 , "z":21700, "rotate":0}
+ ],
+"The Torment" : [
+ {"x":14610 , "z":32310, "rotate":0}
+ ],
+"The Tyros Ridge" : [
+ {"x":17350 , "z":48600, "rotate":0}
+ ],
+"The Vela Molecular Ridge" : [
+ {"x":4750 , "z":-1310, "rotate":0}
+ ],
+"Vela Ultima Complex" : [
+ {"x":20270 , "z":-1210, "rotate":0}
+ ],
+"Via Maris" : [
+ {"x":-13430 , "z":640, "rotate":0}
+ ],
+"Viatori Patuit" : [
+ {"x":28460 , "z":44280, "rotate":0}
+ ],
+"Wagar's Reach" : [
+ {"x":-29190 , "z":32690, "rotate":0}
+ ],
+"Watkuweis" : [
+ {"x":11635 , "z":34290, "rotate":0}
+ ],
+"The Wayfarers Graveyard" : [
+ {"x":-3255 , "z":-3460, "rotate":0}
+ ],
+"Western Neutron Fields" : [
+ {"x":-5330 , "z":25320, "rotate":0}
+ ],
+"Zephyrus" : [
+ {"x":-20790 , "z":41240, "rotate":0}
+ ]
+
+
+ }
+}
\ No newline at end of file
diff --git a/data/milkyway.json b/data/milkyway.json
new file mode 100644
index 0000000..2feb682
--- /dev/null
+++ b/data/milkyway.json
@@ -0,0 +1,131 @@
+{
+
+ "quadrants" : {
+ "The Orion Spur" : {
+ "x":0, "z":0, "rotate":0
+ },
+ "Galactic Core Region" : {
+ "x":974, "z":27333, "rotate":0
+ },
+ "Upper 2nd quadrant" : {
+ "x":-10000, "z":-2000, "rotate":0
+ },
+ "Upper 3rd quadrant" : {
+ "x":10000, "z":-2000, "rotate":0
+ },
+ "Lower 3rd quadrant" : {
+ "x":20000, "z":-12000, "rotate":0
+ },
+ "Lower 1st quadrant" : {
+ "x":-15000, "z":5000, "rotate":0
+ },
+ "Intermediate 1st quadrant" : {
+ "x":-20000, "z":24000, "rotate":0
+ },
+ "Upper 1st quadrant" : {
+ "x":-15000, "z":43000, "rotate":0
+ },
+ "Lower 4th quadrant" : {
+ "x":15000, "z":5000, "rotate":0
+ },
+ "Intermediate 4th quadrant" : {
+ "x":20000, "z":24000, "rotate":0
+ },
+ "Upper 4th quadrant" : {
+ "x":15000, "z":43000, "rotate":0
+ },
+ "The 4th quadrant Tenebris" : {
+ "x":28492, "z":6298, "rotate":45
+ }
+ },
+
+ "arms" : {
+ "The Orion Spur" : [
+ {"x":-16284, "z":16823, "rotate":90},
+ {"x":-7265, "z":4226, "rotate":-35},
+ {"x":6858, "z":-576, "rotate":0}
+ ],
+ "The outer Arm vacuus" : [
+ {"x":10, "z":-14500, "rotate":0}
+ ],
+ "The Sagittarius Arm" : [
+ {"x":5379, "z":4768, "rotate":0}
+ ],
+ "The Carina-Sagittarius Arm" : [
+ {"x":22458, "z":10237, "rotate":45},
+ {"x":29632, "z":25285, "rotate":82},
+ {"x":28962, "z":40634, "rotate":105},
+ {"x":17446, "z":58185, "rotate":-40}
+ ],
+ "The new outer Arm" : [
+ {"x":-31158, "z":16529, "rotate":90},
+ {"x":-28281, "z":3070, "rotate":112},
+ {"x":-18972, "z":-8546, "rotate":-35}
+
+ ],
+ "The Perseus Arm" : [
+ {"x":-19961, "z":30870, "rotate":60 },
+ {"x":-22778, "z":10633, "rotate":110 },
+ {"x":-7525, "z":-3597, "rotate":-25 },
+ {"x":8039, "z":-7019, "rotate":0 },
+ {"x":32905, "z":4565, "rotate":45 }
+ ],
+ "The Norma Arm" : [
+ {"x":7944, "z":17960, "rotate":40 },
+ {"x":13524, "z":29918, "rotate":90 },
+ {"x":7498, "z":40348, "rotate":-35 }
+ ],
+ "The Cygnus Arm" : [
+ {"x":-7724, "z":43523, "rotate":0 },
+ {"x":-22353, "z":37643, "rotate":38 }
+ ],
+ "The Scutum-Centaurus Arm" : [
+ {"x":-33364, "z":46016, "rotate":45 },
+ {"x":-15742, "z":54759, "rotate":0 },
+ {"x":-334, "z":53331, "rotate":-14 },
+ {"x":14300, "z":46140, "rotate":-38 },
+ {"x":19815, "z":31418, "rotate":90 },
+ {"x":16637, "z":17458, "rotate":48 }
+ ],
+ "The near 3 KPC Arm" : [
+ {"x":5765, "z":22639, "rotate":45 }
+ ],
+ "The far 3 KPC Arm" : [
+ {"x":-4906 ,"z":28535, "rotate":45 }
+ ]
+ },
+
+ "gaps" : {
+ "The Sagittarius gap" : [
+ {"x":4794 , "z":1917, "rotate":0},
+ {"x":14702 , "z":2381, "rotate":8}
+ ],
+ "The Perseus gap" : [
+ {"x":-18665 , "z":16893, "rotate":90},
+ {"x":-15402 , "z":8394, "rotate":-52},
+ {"x":-8836 , "z":1370, "rotate":-36},
+ {"x":8574 , "z":-3518, "rotate":0}
+ ],
+ "The Orio-Persean gap" : [
+ {"x":-673 , "z":-2609, "rotate":-15}
+ ],
+ "The Carina-Scutum gap" : [
+ {"x":14535 , "z":54942, "rotate":-35},
+ {"x":22140 , "z":45532, "rotate":-60}
+ ]
+
+ },
+
+ "others" : {
+ "Sagittarius A*" : [
+ {"x":25 , "z":25900, "rotate":0}
+ ],
+ "The western neutron fields" : [
+ {"x":-3669 , "z":25478, "rotate":45}
+ ],
+ "The eastern neutron fields" : [
+ {"x":3263 , "z":25727, "rotate":45}
+ ]
+
+ }
+}
\ No newline at end of file
diff --git a/data/spectral-colors.json b/data/spectral-colors.json
new file mode 100644
index 0000000..bc40747
--- /dev/null
+++ b/data/spectral-colors.json
@@ -0,0 +1,19 @@
+{
+ "D":"9bb2fe",
+ "O":"98b4fe",
+ "B":"a0bfff",
+ "A":"d0dafe",
+ "F":"f5f3ff",
+ "G":"fef3ef",
+ "K":"ffe4cf",
+ "M":"ffd1a3",
+ "R":"ffb36b",
+ "N":"ff8913",
+ "L":"f6392b",
+ "T":"aa2c51",
+ "Y":"5d347e",
+ "HERBIG Ae/Be":"9fadb6",
+ "WR":"Ca594b,d3dceb",
+ "S":"cd6136",
+ "C":"cd6136"
+}
\ No newline at end of file
diff --git a/data/station_stats.json b/data/station_stats.json
new file mode 100644
index 0000000..c7ca20c
--- /dev/null
+++ b/data/station_stats.json
@@ -0,0 +1,14727 @@
+[
+ {
+ "title": "Canopus - Puppis Sector CQ-Y b1 (260Ly)",
+ "prison": {
+ "name": "Puppis Sector CQ-Y b1",
+ "coords": {
+ "x": 53.65625,
+ "y": 3.15625,
+ "z": -46.5
+ }
+ },
+ "jump": {
+ "name": "Canopus",
+ "coords": {
+ "x": 276.625,
+ "y": -131.34375,
+ "z": -42.4375
+ }
+ }
+ },
+ {
+ "title": "HIP 18238 - California Sector CQ-Y c5 (161Ly)",
+ "prison": {
+ "name": "California Sector CQ-Y c5",
+ "coords": {
+ "x": -350.375,
+ "y": -245.53125,
+ "z": -950.625
+ }
+ },
+ "jump": {
+ "name": "HIP 18238",
+ "coords": {
+ "x": -324.3125,
+ "y": -171.75,
+ "z": -809.46875
+ }
+ }
+ },
+ {
+ "title": "2MASS J03291977+3124572 - California Sector CQ-Y c5 (140Ly)",
+ "prison": {
+ "name": "California Sector CQ-Y c5",
+ "coords": {
+ "x": -350.375,
+ "y": -245.53125,
+ "z": -950.625
+ }
+ },
+ "jump": {
+ "name": "2MASS J03291977+3124572",
+ "coords": {
+ "x": -379.8125,
+ "y": -382.09375,
+ "z": -954.46875
+ }
+ }
+ },
+ {
+ "title": "HR 1185 - Pleiades Sector HR-W d1-79 (21Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "HR 1185",
+ "coords": {
+ "x": -64.65625,
+ "y": -148.9375,
+ "z": -330.40625
+ }
+ }
+ },
+ {
+ "title": "HIP 83506 - Col 285 Sector YU-F c11-1 (215Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 83506",
+ "coords": {
+ "x": 125.46875,
+ "y": -36.8125,
+ "z": 303.46875
+ }
+ }
+ },
+ {
+ "title": "New Yembo - G 203-51 (326Ly)",
+ "prison": {
+ "name": "G 203-51",
+ "coords": {
+ "x": -15.875,
+ "y": 12.03125,
+ "z": 5.34375
+ }
+ },
+ "jump": {
+ "name": "New Yembo",
+ "coords": {
+ "x": -334.625,
+ "y": -4.46875,
+ "z": 73.5
+ }
+ }
+ },
+ {
+ "title": "Mammon - Capricorni Sector FM-V b2-0 (879Ly)",
+ "prison": {
+ "name": "Capricorni Sector FM-V b2-0",
+ "coords": {
+ "x": -103.59375,
+ "y": -100.375,
+ "z": 97.4375
+ }
+ },
+ "jump": {
+ "name": "Mammon",
+ "coords": {
+ "x": -358.375,
+ "y": -8.75,
+ "z": 933.53125
+ }
+ }
+ },
+ {
+ "title": "Taygeta - Pleiades Sector HR-W d1-79 (28Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Taygeta",
+ "coords": {
+ "x": -88.5,
+ "y": -159.6875,
+ "z": -366.28125
+ }
+ }
+ },
+ {
+ "title": "Maia - Pleiades Sector HR-W d1-79 (3Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Maia",
+ "coords": {
+ "x": -81.78125,
+ "y": -149.4375,
+ "z": -343.375
+ }
+ }
+ },
+ {
+ "title": "HIP 8593 - Col 285 Sector YU-F c11-1 (156Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 8593",
+ "coords": {
+ "x": 107.46875,
+ "y": -226.8125,
+ "z": 18.53125
+ }
+ }
+ },
+ {
+ "title": "GM Cephei - California Sector CQ-Y c5 (2406Ly)",
+ "prison": {
+ "name": "California Sector CQ-Y c5",
+ "coords": {
+ "x": -350.375,
+ "y": -245.53125,
+ "z": -950.625
+ }
+ },
+ "jump": {
+ "name": "GM Cephei",
+ "coords": {
+ "x": -2660.96875,
+ "y": 180.15625,
+ "z": -433.15625
+ }
+ }
+ },
+ {
+ "title": "HIP 58832 - Col 285 Sector YG-Y b16-1 (5300Ly)",
+ "prison": {
+ "name": "Col 285 Sector YG-Y b16-1",
+ "coords": {
+ "x": 16.78125,
+ "y": 138.84375,
+ "z": -2.5
+ }
+ },
+ "jump": {
+ "name": "HIP 58832",
+ "coords": {
+ "x": -111.6875,
+ "y": 5319.21875,
+ "z": -1115.375
+ }
+ }
+ },
+ {
+ "title": "HIP 11144 - ICZ KS-T b3-5 (176Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 11144",
+ "coords": {
+ "x": 98.65625,
+ "y": -260.21875,
+ "z": -41.90625
+ }
+ }
+ },
+ {
+ "title": "HIP 6949 - HR 32 (103Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 6949",
+ "coords": {
+ "x": 104.84375,
+ "y": -233.71875,
+ "z": 34.375
+ }
+ }
+ },
+ {
+ "title": "HIP 293 - Col 285 Sector YU-F c11-1 (140Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 293",
+ "coords": {
+ "x": 98.0,
+ "y": -232.25,
+ "z": 98.0
+ }
+ }
+ },
+ {
+ "title": "HIP 15614 - HR 32 (73Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 15614",
+ "coords": {
+ "x": 182.90625,
+ "y": -177.71875,
+ "z": 49.0
+ }
+ }
+ },
+ {
+ "title": "HIP 5549 - HR 32 (47Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 5549",
+ "coords": {
+ "x": 175.65625,
+ "y": -144.25,
+ "z": 110.28125
+ }
+ }
+ },
+ {
+ "title": "HIP 10570 - HR 32 (118Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 10570",
+ "coords": {
+ "x": 139.78125,
+ "y": -242.03125,
+ "z": 16.09375
+ }
+ }
+ },
+ {
+ "title": "HIP 23759 - Pleiades Sector HR-W d1-79 (626Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "HIP 23759",
+ "coords": {
+ "x": 359.84375,
+ "y": -385.53125,
+ "z": -718.375
+ }
+ }
+ },
+ {
+ "title": "HIP 18390 - California Sector CQ-Y c5 (92Ly)",
+ "prison": {
+ "name": "California Sector CQ-Y c5",
+ "coords": {
+ "x": -350.375,
+ "y": -245.53125,
+ "z": -950.625
+ }
+ },
+ "jump": {
+ "name": "HIP 18390",
+ "coords": {
+ "x": -299.0625,
+ "y": -229.25,
+ "z": -876.125
+ }
+ }
+ },
+ {
+ "title": "HIP 17519 - Hyades Sector LC-L b8-0 (152Ly)",
+ "prison": {
+ "name": "Hyades Sector LC-L b8-0",
+ "coords": {
+ "x": 93.71875,
+ "y": -103.625,
+ "z": -85.9375
+ }
+ },
+ "jump": {
+ "name": "HIP 17519",
+ "coords": {
+ "x": 17.375,
+ "y": -160.84375,
+ "z": -204.5
+ }
+ }
+ },
+ {
+ "title": "Beta Horologii - Synuefe BR-L c24-24 (108Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Beta Horologii",
+ "coords": {
+ "x": 192.96875,
+ "y": -218.46875,
+ "z": 42.90625
+ }
+ }
+ },
+ {
+ "title": "LTT 9846 - ICZ ZK-X b1-0 (170Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "LTT 9846",
+ "coords": {
+ "x": -89.84375,
+ "y": -271.96875,
+ "z": 11.09375
+ }
+ }
+ },
+ {
+ "title": "HIP 16753 - Pleiades Sector HR-W d1-79 (28Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "HIP 16753",
+ "coords": {
+ "x": -88.3125,
+ "y": -172.9375,
+ "z": -348.84375
+ }
+ }
+ },
+ {
+ "title": "HIP 11459 - ICZ KS-T b3-5 (178Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 11459",
+ "coords": {
+ "x": 98.25,
+ "y": -258.5625,
+ "z": -47.90625
+ }
+ }
+ },
+ {
+ "title": "HIP 7060 - Col 285 Sector YU-F c11-1 (161Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 7060",
+ "coords": {
+ "x": 107.34375,
+ "y": -240.125,
+ "z": 33.59375
+ }
+ }
+ },
+ {
+ "title": "HIP 494 - Col 285 Sector YU-F c11-1 (102Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 494",
+ "coords": {
+ "x": 132.09375,
+ "y": -188.9375,
+ "z": 106.21875
+ }
+ }
+ },
+ {
+ "title": "HIP 596 - HR 32 (34Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 596",
+ "coords": {
+ "x": 160.1875,
+ "y": -168.90625,
+ "z": 116.9375
+ }
+ }
+ },
+ {
+ "title": "HIP 74290 - Col 285 Sector YU-F c11-1 (245Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 74290",
+ "coords": {
+ "x": 254.34375,
+ "y": -39.0625,
+ "z": 278.59375
+ }
+ }
+ },
+ {
+ "title": "Aditi - ICZ KS-T b3-5 (291Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Aditi",
+ "coords": {
+ "x": 72.875,
+ "y": -337.84375,
+ "z": -159.40625
+ }
+ }
+ },
+ {
+ "title": "HIP 16813 - Pleiades Sector HR-W d1-79 (79Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "HIP 16813",
+ "coords": {
+ "x": -57.03125,
+ "y": -143.375,
+ "z": -268.28125
+ }
+ }
+ },
+ {
+ "title": "HIP 791 - ICZ KS-T b3-5 (106Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 791",
+ "coords": {
+ "x": 32.5625,
+ "y": -241.53125,
+ "z": 59.625
+ }
+ }
+ },
+ {
+ "title": "HIP 11493 - Hyades Sector LC-L b8-0 (141Ly)",
+ "prison": {
+ "name": "Hyades Sector LC-L b8-0",
+ "coords": {
+ "x": 93.71875,
+ "y": -103.625,
+ "z": -85.9375
+ }
+ },
+ "jump": {
+ "name": "HIP 11493",
+ "coords": {
+ "x": 105.0,
+ "y": -230.53125,
+ "z": -25.875
+ }
+ }
+ },
+ {
+ "title": "BD+22 4939 - Arietis Sector ZF-O b6-1 (172Ly)",
+ "prison": {
+ "name": "Arietis Sector ZF-O b6-1",
+ "coords": {
+ "x": -89.53125,
+ "y": -102.4375,
+ "z": -75.4375
+ }
+ },
+ "jump": {
+ "name": "BD+22 4939",
+ "coords": {
+ "x": -235.90625,
+ "y": -192.84375,
+ "z": -80.46875
+ }
+ }
+ },
+ {
+ "title": "Takurua - Pleiades Sector HR-W d1-79 (241Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Takurua",
+ "coords": {
+ "x": -253.8125,
+ "y": -4.0625,
+ "z": -254.1875
+ }
+ }
+ },
+ {
+ "title": "HIP 8396 - Lyncis Sector QJ-Q b5-2 (194Ly)",
+ "prison": {
+ "name": "Lyncis Sector QJ-Q b5-2",
+ "coords": {
+ "x": -99.75,
+ "y": 100.53125,
+ "z": -99.21875
+ }
+ },
+ "jump": {
+ "name": "HIP 8396",
+ "coords": {
+ "x": -226.5,
+ "y": -12.46875,
+ "z": -193.5
+ }
+ }
+ },
+ {
+ "title": "HIP 17044 - Pleiades Sector HR-W d1-79 (63Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "HIP 17044",
+ "coords": {
+ "x": -74.25,
+ "y": -129.53125,
+ "z": -283.15625
+ }
+ }
+ },
+ {
+ "title": "HIP 1742 - ICZ KS-T b3-5 (106Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 1742",
+ "coords": {
+ "x": 29.1875,
+ "y": -244.46875,
+ "z": 46.28125
+ }
+ }
+ },
+ {
+ "title": "HIP 118045 - ICZ KS-T b3-5 (113Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 118045",
+ "coords": {
+ "x": 43.28125,
+ "y": -240.21875,
+ "z": 77.21875
+ }
+ }
+ },
+ {
+ "title": "HIP 10325 - ICZ KS-T b3-5 (149Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 10325",
+ "coords": {
+ "x": 89.875,
+ "y": -237.5625,
+ "z": -25.1875
+ }
+ }
+ },
+ {
+ "title": "HIP 1781 - Col 285 Sector YU-F c11-1 (172Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 1781",
+ "coords": {
+ "x": 91.625,
+ "y": -263.65625,
+ "z": 83.96875
+ }
+ }
+ },
+ {
+ "title": "HIP 7154 - Col 285 Sector YU-F c11-1 (151Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 7154",
+ "coords": {
+ "x": 105.65625,
+ "y": -229.21875,
+ "z": 33.5
+ }
+ }
+ },
+ {
+ "title": "Laka - Col 285 Sector YU-F c11-1 (143Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Laka",
+ "coords": {
+ "x": 110.40625,
+ "y": -234.53125,
+ "z": 115.09375
+ }
+ }
+ },
+ {
+ "title": "HIP 17269 - HR 32 (108Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 17269",
+ "coords": {
+ "x": 171.8125,
+ "y": -185.21875,
+ "z": 2.21875
+ }
+ }
+ },
+ {
+ "title": "Dilgaenses - HR 32 (103Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Dilgaenses",
+ "coords": {
+ "x": 135.6875,
+ "y": -220.09375,
+ "z": 15.9375
+ }
+ }
+ },
+ {
+ "title": "Xakria - HR 32 (48Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Xakria",
+ "coords": {
+ "x": 138.40625,
+ "y": -198.28125,
+ "z": 72.53125
+ }
+ }
+ },
+ {
+ "title": "Gamma-3 Octantis - Col 285 Sector YU-F c11-1 (93Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Gamma-3 Octantis",
+ "coords": {
+ "x": 171.53125,
+ "y": -146.25,
+ "z": 118.34375
+ }
+ }
+ },
+ {
+ "title": "HIP 9610 - HR 32 (45Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 9610",
+ "coords": {
+ "x": 176.9375,
+ "y": -160.625,
+ "z": 94.5625
+ }
+ }
+ },
+ {
+ "title": "HIP 25759 - Synuefe BR-L c24-24 (24Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 25759",
+ "coords": {
+ "x": 210.625,
+ "y": -137.59375,
+ "z": 6.125
+ }
+ }
+ },
+ {
+ "title": "HIP 26174 - Pleiades Sector HR-W d1-79 (482Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "HIP 26174",
+ "coords": {
+ "x": 297.5,
+ "y": -212.59375,
+ "z": -635.25
+ }
+ }
+ },
+ {
+ "title": "HIP 17892 - Pleiades Sector HR-W d1-79 (18Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "HIP 17892",
+ "coords": {
+ "x": -70.75,
+ "y": -157.9375,
+ "z": -352.84375
+ }
+ }
+ },
+ {
+ "title": "HIP 116510 - ICZ ZK-X b1-0 (148Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "HIP 116510",
+ "coords": {
+ "x": 37.21875,
+ "y": -235.84375,
+ "z": 91.28125
+ }
+ }
+ },
+ {
+ "title": "17 Draconis - G 203-51 (392Ly)",
+ "prison": {
+ "name": "G 203-51",
+ "coords": {
+ "x": -15.875,
+ "y": 12.03125,
+ "z": 5.34375
+ }
+ },
+ "jump": {
+ "name": "17 Draconis",
+ "coords": {
+ "x": -305.75,
+ "y": 272.21875,
+ "z": 49.5
+ }
+ }
+ },
+ {
+ "title": "HIP 10792 - Pleiades Sector HR-W d1-79 (334Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "HIP 10792",
+ "coords": {
+ "x": -366.09375,
+ "y": 26.5,
+ "z": -337.65625
+ }
+ }
+ },
+ {
+ "title": "HIP 17225 - Pleiades Sector HR-W d1-79 (24Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "HIP 17225",
+ "coords": {
+ "x": -86.15625,
+ "y": -165.59375,
+ "z": -356.8125
+ }
+ }
+ },
+ {
+ "title": "HIP 11575 - HR 32 (164Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 11575",
+ "coords": {
+ "x": 116.84375,
+ "y": -257.6875,
+ "z": -30.78125
+ }
+ }
+ },
+ {
+ "title": "Seagull Sector DL-Y d3 - HR 3005 (3197Ly)",
+ "prison": {
+ "name": "HR 3005",
+ "coords": {
+ "x": 750.53125,
+ "y": -169.65625,
+ "z": -90
+ }
+ },
+ "jump": {
+ "name": "Seagull Sector DL-Y d3",
+ "coords": {
+ "x": 2608.15625,
+ "y": -181.96875,
+ "z": -2692.28125
+ }
+ }
+ },
+ {
+ "title": "HR 8019 - HR 32 (74Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HR 8019",
+ "coords": {
+ "x": 115.40625,
+ "y": -158.0,
+ "z": 171.375
+ }
+ }
+ },
+ {
+ "title": "HR 8662 - Col 285 Sector YU-F c11-1 (146Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HR 8662",
+ "coords": {
+ "x": 32.71875,
+ "y": -219.375,
+ "z": 127.0625
+ }
+ }
+ },
+ {
+ "title": "42 n Persei - Pleiades Sector HR-W d1-79 (123Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "42 n Persei",
+ "coords": {
+ "x": -83.5625,
+ "y": -73.40625,
+ "z": -244.34375
+ }
+ }
+ },
+ {
+ "title": "HIP 509 - ICZ ZK-X b1-0 (137Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "HIP 509",
+ "coords": {
+ "x": -5.59375,
+ "y": -254.59375,
+ "z": 45.34375
+ }
+ }
+ },
+ {
+ "title": "Fehu - ICZ KS-T b3-5 (353Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Fehu",
+ "coords": {
+ "x": 46.375,
+ "y": -448.6875,
+ "z": -127.125
+ }
+ }
+ },
+ {
+ "title": "HIP 1795 - ICZ KS-T b3-5 (111Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 1795",
+ "coords": {
+ "x": 32.125,
+ "y": -248.0625,
+ "z": 47.6875
+ }
+ }
+ },
+ {
+ "title": "HIP 1567 - Col 285 Sector YU-F c11-1 (133Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 1567",
+ "coords": {
+ "x": 84.40625,
+ "y": -223.25,
+ "z": 77.375
+ }
+ }
+ },
+ {
+ "title": "HIP 12248 - HR 32 (143Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 12248",
+ "coords": {
+ "x": 112.6875,
+ "y": -224.8125,
+ "z": -26.40625
+ }
+ }
+ },
+ {
+ "title": "HIP 62154 - Crucis Sector ND-S b4-5 (382Ly)",
+ "prison": {
+ "name": "Crucis Sector ND-S b4-5",
+ "coords": {
+ "x": 78.6875,
+ "y": 53.9375,
+ "z": 80.03125
+ }
+ },
+ "jump": {
+ "name": "HIP 62154",
+ "coords": {
+ "x": 411.375,
+ "y": 9.09375,
+ "z": 261.46875
+ }
+ }
+ },
+ {
+ "title": "T Tauri - Pleiades Sector HR-W d1-79 (227Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "T Tauri",
+ "coords": {
+ "x": -32.96875,
+ "y": -206.40625,
+ "z": -557.3125
+ }
+ }
+ },
+ {
+ "title": "HIP 1977 - Col 285 Sector YU-F c11-1 (156Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 1977",
+ "coords": {
+ "x": 83.375,
+ "y": -246.3125,
+ "z": 75.09375
+ }
+ }
+ },
+ {
+ "title": "HIP 1419 - Col 285 Sector YU-F c11-1 (119Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 1419",
+ "coords": {
+ "x": 132.75,
+ "y": -206.0,
+ "z": 103.5
+ }
+ }
+ },
+ {
+ "title": "HIP 20920 - HR 32 (92Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 20920",
+ "coords": {
+ "x": 205.5,
+ "y": -161.15625,
+ "z": 43.34375
+ }
+ }
+ },
+ {
+ "title": "HIP 15886 - Synuefe BR-L c24-24 (100Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 15886",
+ "coords": {
+ "x": 190.8125,
+ "y": -157.59375,
+ "z": 79.3125
+ }
+ }
+ },
+ {
+ "title": "Pencil Sector EL-Y d5 - HR 3005 (189Ly)",
+ "prison": {
+ "name": "HR 3005",
+ "coords": {
+ "x": 750.53125,
+ "y": -169.65625,
+ "z": -90
+ }
+ },
+ "jump": {
+ "name": "Pencil Sector EL-Y d5",
+ "coords": {
+ "x": 816.3125,
+ "y": 2.0,
+ "z": -44.03125
+ }
+ }
+ },
+ {
+ "title": "Celaeno - Pleiades Sector HR-W d1-79 (6Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Celaeno",
+ "coords": {
+ "x": -81.09375,
+ "y": -148.3125,
+ "z": -337.09375
+ }
+ }
+ },
+ {
+ "title": "Electra - Pleiades Sector HR-W d1-79 (23Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Electra",
+ "coords": {
+ "x": -86.0625,
+ "y": -159.9375,
+ "z": -361.65625
+ }
+ }
+ },
+ {
+ "title": "Asterope - Pleiades Sector HR-W d1-79 (10Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Asterope",
+ "coords": {
+ "x": -80.15625,
+ "y": -144.09375,
+ "z": -333.375
+ }
+ }
+ },
+ {
+ "title": "HIP 8807 - ICZ KS-T b3-5 (142Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 8807",
+ "coords": {
+ "x": 55.8125,
+ "y": -247.25,
+ "z": -34.625
+ }
+ }
+ },
+ {
+ "title": "HIP 8193 - HR 32 (84Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 8193",
+ "coords": {
+ "x": 121.03125,
+ "y": -216.0,
+ "z": 38.0625
+ }
+ }
+ },
+ {
+ "title": "HIP 1536 - HR 32 (57Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 1536",
+ "coords": {
+ "x": 144.875,
+ "y": -214.03125,
+ "z": 110.71875
+ }
+ }
+ },
+ {
+ "title": "Merope - Pleiades Sector HR-W d1-79 (5Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Merope",
+ "coords": {
+ "x": -78.59375,
+ "y": -149.625,
+ "z": -340.53125
+ }
+ }
+ },
+ {
+ "title": "HIP 17692 - Pleiades Sector HR-W d1-79 (16Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "HIP 17692",
+ "coords": {
+ "x": -74.125,
+ "y": -143.96875,
+ "z": -328.78125
+ }
+ }
+ },
+ {
+ "title": "HIP 17694 - Pleiades Sector HR-W d1-79 (11Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "HIP 17694",
+ "coords": {
+ "x": -72.25,
+ "y": -152.53125,
+ "z": -338.6875
+ }
+ }
+ },
+ {
+ "title": "Soul Sector EL-Y d7 - California Sector CQ-Y c5 (6553Ly)",
+ "prison": {
+ "name": "California Sector CQ-Y c5",
+ "coords": {
+ "x": -350.375,
+ "y": -245.53125,
+ "z": -950.625
+ }
+ },
+ "jump": {
+ "name": "Soul Sector EL-Y d7",
+ "coords": {
+ "x": -5043.15625,
+ "y": 85.03125,
+ "z": -5513.09375
+ }
+ }
+ },
+ {
+ "title": "HR 1172 - Pleiades Sector HR-W d1-79 (9Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "HR 1172",
+ "coords": {
+ "x": -75.1875,
+ "y": -152.40625,
+ "z": -346.59375
+ }
+ }
+ },
+ {
+ "title": "HIP 1185 - ICZ ZK-X b1-0 (172Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "HIP 1185",
+ "coords": {
+ "x": -77.1875,
+ "y": -279.46875,
+ "z": -0.3125
+ }
+ }
+ },
+ {
+ "title": "HIP 12965 - HR 32 (159Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 12965",
+ "coords": {
+ "x": 113.9375,
+ "y": -231.28125,
+ "z": -41.5625
+ }
+ }
+ },
+ {
+ "title": "HIP 8251 - ICZ ZK-X b1-0 (159Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "HIP 8251",
+ "coords": {
+ "x": 97.96875,
+ "y": -237.78125,
+ "z": 11.84375
+ }
+ }
+ },
+ {
+ "title": "Atlas - Pleiades Sector HR-W d1-79 (4Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Atlas",
+ "coords": {
+ "x": -76.71875,
+ "y": -147.34375,
+ "z": -344.4375
+ }
+ }
+ },
+ {
+ "title": "Pleione - Pleiades Sector HR-W d1-79 (4Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Pleione",
+ "coords": {
+ "x": -77.0,
+ "y": -146.78125,
+ "z": -344.125
+ }
+ }
+ },
+ {
+ "title": "HR 1183 - Pleiades Sector HR-W d1-79 (10Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "HR 1183",
+ "coords": {
+ "x": -72.875,
+ "y": -145.09375,
+ "z": -336.96875
+ }
+ }
+ },
+ {
+ "title": "HIP 112001 - ICZ ZK-X b1-0 (169Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "HIP 112001",
+ "coords": {
+ "x": 8.34375,
+ "y": -236.3125,
+ "z": 134.0625
+ }
+ }
+ },
+ {
+ "title": "HIP 9146 - ICZ ZK-X b1-0 (152Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "HIP 9146",
+ "coords": {
+ "x": 60.84375,
+ "y": -247.09375,
+ "z": -36.21875
+ }
+ }
+ },
+ {
+ "title": "HIP 2574 - ICZ KS-T b3-5 (122Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 2574",
+ "coords": {
+ "x": 22.84375,
+ "y": -261.6875,
+ "z": 33.125
+ }
+ }
+ },
+ {
+ "title": "HIP 17497 - Pleiades Sector HR-W d1-79 (13Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "HIP 17497",
+ "coords": {
+ "x": -79.125,
+ "y": -158.6875,
+ "z": -349.125
+ }
+ }
+ },
+ {
+ "title": "HIP 22460 - Pleiades Sector HR-W d1-79 (97Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "HIP 22460",
+ "coords": {
+ "x": -41.3125,
+ "y": -58.96875,
+ "z": -354.78125
+ }
+ }
+ },
+ {
+ "title": "HIP 8711 - HR 32 (119Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 8711",
+ "coords": {
+ "x": 148.75,
+ "y": -261.53125,
+ "z": 41.1875
+ }
+ }
+ },
+ {
+ "title": "HIP 2761 - HR 32 (96Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 2761",
+ "coords": {
+ "x": 131.375,
+ "y": -255.65625,
+ "z": 96.21875
+ }
+ }
+ },
+ {
+ "title": "Crab Sector DL-Y d9 - California Sector CQ-Y c5 (6083Ly)",
+ "prison": {
+ "name": "California Sector CQ-Y c5",
+ "coords": {
+ "x": -350.375,
+ "y": -245.53125,
+ "z": -950.625
+ }
+ },
+ "jump": {
+ "name": "Crab Sector DL-Y d9",
+ "coords": {
+ "x": 559.625,
+ "y": -708.0625,
+ "z": -6947.5625
+ }
+ }
+ },
+ {
+ "title": "Amatsuboshi - Eol Prou LW-L c8-127 (15Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Amatsuboshi",
+ "coords": {
+ "x": -9516.03125,
+ "y": -908.25,
+ "z": 19802.71875
+ }
+ }
+ },
+ {
+ "title": "Skaude DR-A c2-1 - Nyeajaae VU-Y a27-9 (2709Ly)",
+ "prison": {
+ "name": "Nyeajaae VU-Y a27-9",
+ "coords": {
+ "x": -3374,
+ "y": -42.375,
+ "z": 6907.96875
+ }
+ },
+ "jump": {
+ "name": "Skaude DR-A c2-1",
+ "coords": {
+ "x": -4661.15625,
+ "y": -420.0,
+ "z": 9261.40625
+ }
+ }
+ },
+ {
+ "title": "HIP 1290 - ICZ KS-T b3-5 (115Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 1290",
+ "coords": {
+ "x": -15.5,
+ "y": -257.1875,
+ "z": 28.96875
+ }
+ }
+ },
+ {
+ "title": "HIP 13864 - Hyades Sector LC-L b8-0 (128Ly)",
+ "prison": {
+ "name": "Hyades Sector LC-L b8-0",
+ "coords": {
+ "x": 93.71875,
+ "y": -103.625,
+ "z": -85.9375
+ }
+ },
+ "jump": {
+ "name": "HIP 13864",
+ "coords": {
+ "x": 114.84375,
+ "y": -224.96875,
+ "z": -52.875
+ }
+ }
+ },
+ {
+ "title": "HIP 3084 - HR 32 (71Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 3084",
+ "coords": {
+ "x": 106.5625,
+ "y": -221.375,
+ "z": 76.15625
+ }
+ }
+ },
+ {
+ "title": "FW Cephei - California Sector CQ-Y c5 (1365Ly)",
+ "prison": {
+ "name": "California Sector CQ-Y c5",
+ "coords": {
+ "x": -350.375,
+ "y": -245.53125,
+ "z": -950.625
+ }
+ },
+ "jump": {
+ "name": "FW Cephei",
+ "coords": {
+ "x": -1415.78125,
+ "y": 366.65625,
+ "z": -355.3125
+ }
+ }
+ },
+ {
+ "title": "Yukilam - ICZ KS-T b3-5 (150Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Yukilam",
+ "coords": {
+ "x": 93.40625,
+ "y": -235.96875,
+ "z": -25.84375
+ }
+ }
+ },
+ {
+ "title": "Agulu - Col 285 Sector YU-F c11-1 (155Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Agulu",
+ "coords": {
+ "x": 93.3125,
+ "y": -242.46875,
+ "z": 60.0
+ }
+ }
+ },
+ {
+ "title": "Quince - Hydrae Sector ON-T b3-1 (358Ly)",
+ "prison": {
+ "name": "Hydrae Sector ON-T b3-1",
+ "coords": {
+ "x": 99.875,
+ "y": 92.96875,
+ "z": 78.0625
+ }
+ },
+ "jump": {
+ "name": "Quince",
+ "coords": {
+ "x": 57.375,
+ "y": 341.375,
+ "z": 333.0
+ }
+ }
+ },
+ {
+ "title": "HIP 7395 - HR 32 (128Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 7395",
+ "coords": {
+ "x": 104.21875,
+ "y": -258.59375,
+ "z": 22.8125
+ }
+ }
+ },
+ {
+ "title": "Kising - HR 32 (114Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Kising",
+ "coords": {
+ "x": 100.15625,
+ "y": -260.8125,
+ "z": 59.03125
+ }
+ }
+ },
+ {
+ "title": "Arakates - Synuefe BR-L c24-24 (91Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Arakates",
+ "coords": {
+ "x": 167.0,
+ "y": -186.5625,
+ "z": -44.125
+ }
+ }
+ },
+ {
+ "title": "Papako - HR 32 (65Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Papako",
+ "coords": {
+ "x": 141.1875,
+ "y": -218.34375,
+ "z": 124.78125
+ }
+ }
+ },
+ {
+ "title": "Cantzicnal - Synuefe BR-L c24-24 (62Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Cantzicnal",
+ "coords": {
+ "x": 192.875,
+ "y": -177.6875,
+ "z": 8.5
+ }
+ }
+ },
+ {
+ "title": "Scirians - HR 32 (47Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Scirians",
+ "coords": {
+ "x": 178.75,
+ "y": -164.59375,
+ "z": 94.0
+ }
+ }
+ },
+ {
+ "title": "HIP 112123 - ICZ ZK-X b1-0 (154Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "HIP 112123",
+ "coords": {
+ "x": 15.71875,
+ "y": -220.28125,
+ "z": 125.9375
+ }
+ }
+ },
+ {
+ "title": "HIP 14116 - HR 32 (145Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 14116",
+ "coords": {
+ "x": 126.53125,
+ "y": -214.21875,
+ "z": -35.28125
+ }
+ }
+ },
+ {
+ "title": "HIP 9051 - Synuefe BR-L c24-24 (146Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 9051",
+ "coords": {
+ "x": 151.75,
+ "y": -242.09375,
+ "z": 46.0625
+ }
+ }
+ },
+ {
+ "title": "NGC 7822 Sector BQ-Y d12 - California Sector CQ-Y c5 (2205Ly)",
+ "prison": {
+ "name": "California Sector CQ-Y c5",
+ "coords": {
+ "x": -350.375,
+ "y": -245.53125,
+ "z": -950.625
+ }
+ },
+ "jump": {
+ "name": "NGC 7822 Sector BQ-Y d12",
+ "coords": {
+ "x": -2454.1875,
+ "y": 299.0625,
+ "z": -1326.0625
+ }
+ }
+ },
+ {
+ "title": "HIP 3020 - HR 32 (122Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 3020",
+ "coords": {
+ "x": 65.6875,
+ "y": -250.09375,
+ "z": 52.71875
+ }
+ }
+ },
+ {
+ "title": "HIP 3488 - Col 285 Sector YU-F c11-1 (112Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 3488",
+ "coords": {
+ "x": 152.0625,
+ "y": -190.40625,
+ "z": 100.71875
+ }
+ }
+ },
+ {
+ "title": "HIP 9768 - Synuefe BR-L c24-24 (155Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 9768",
+ "coords": {
+ "x": 134.84375,
+ "y": -251.09375,
+ "z": 18.78125
+ }
+ }
+ },
+ {
+ "title": "HIP 4062 - Col 285 Sector YU-F c11-1 (120Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 4062",
+ "coords": {
+ "x": 171.625,
+ "y": -187.1875,
+ "z": 110.3125
+ }
+ }
+ },
+ {
+ "title": "HIP 9846 - HR 32 (144Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 9846",
+ "coords": {
+ "x": 119.5625,
+ "y": -261.875,
+ "z": -0.75
+ }
+ }
+ },
+ {
+ "title": "HIP 97024 - Col 285 Sector YU-F c11-1 (90Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 97024",
+ "coords": {
+ "x": 134.96875,
+ "y": -126.46875,
+ "z": 172.0625
+ }
+ }
+ },
+ {
+ "title": "HIP 24968 - Synuefe BR-L c24-24 (25Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 24968",
+ "coords": {
+ "x": 219.71875,
+ "y": -151.78125,
+ "z": -16.84375
+ }
+ }
+ },
+ {
+ "title": "HIP 19072 - Bei Dou Sector DL-Y d76 (364Ly)",
+ "prison": {
+ "name": "Bei Dou Sector DL-Y d76",
+ "coords": {
+ "x": -37.6875,
+ "y": 70.09375,
+ "z": -14.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 19072",
+ "coords": {
+ "x": -54.28125,
+ "y": -121.03125,
+ "z": -323.0625
+ }
+ }
+ },
+ {
+ "title": "Iota Tucanae - HR 32 (94Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Iota Tucanae",
+ "coords": {
+ "x": 150.5,
+ "y": -250.71875,
+ "z": 84.03125
+ }
+ }
+ },
+ {
+ "title": "HIP 24986 - Synuefe BR-L c24-24 (31Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 24986",
+ "coords": {
+ "x": 217.40625,
+ "y": -146.875,
+ "z": 15.3125
+ }
+ }
+ },
+ {
+ "title": "HIP 5488 - HR 32 (90Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 5488",
+ "coords": {
+ "x": 122.28125,
+ "y": -240.90625,
+ "z": 62.65625
+ }
+ }
+ },
+ {
+ "title": "HIP 10941 - Synuefe BR-L c24-24 (111Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 10941",
+ "coords": {
+ "x": 169.65625,
+ "y": -178.03125,
+ "z": 72.5625
+ }
+ }
+ },
+ {
+ "title": "HIP 25879 - Synuefe BR-L c24-24 (27Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 25879",
+ "coords": {
+ "x": 221.90625,
+ "y": -143.6875,
+ "z": 14.0
+ }
+ }
+ },
+ {
+ "title": "HIP 6108 - ICZ KS-T b3-5 (131Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 6108",
+ "coords": {
+ "x": 0.71875,
+ "y": -248.875,
+ "z": -34.84375
+ }
+ }
+ },
+ {
+ "title": "HIP 10754 - Synuefe BR-L c24-24 (121Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 10754",
+ "coords": {
+ "x": 162.21875,
+ "y": -212.0,
+ "z": 49.9375
+ }
+ }
+ },
+ {
+ "title": "HIP 26144 - Synuefe BR-L c24-24 (17Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 26144",
+ "coords": {
+ "x": 214.5,
+ "y": -137.8125,
+ "z": -4.3125
+ }
+ }
+ },
+ {
+ "title": "HIP 24084 - HR 32 (81Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 24084",
+ "coords": {
+ "x": 209.4375,
+ "y": -136.6875,
+ "z": 88.125
+ }
+ }
+ },
+ {
+ "title": "Shenve - Bei Dou Sector DL-Y d76 (913Ly)",
+ "prison": {
+ "name": "Bei Dou Sector DL-Y d76",
+ "coords": {
+ "x": -37.6875,
+ "y": 70.09375,
+ "z": -14.1875
+ }
+ },
+ "jump": {
+ "name": "Shenve",
+ "coords": {
+ "x": 351.96875,
+ "y": -373.46875,
+ "z": -711.09375
+ }
+ }
+ },
+ {
+ "title": "Skaudai AO-V b47-0 - Nyeajaae VU-Y a27-9 (3861Ly)",
+ "prison": {
+ "name": "Nyeajaae VU-Y a27-9",
+ "coords": {
+ "x": -3374,
+ "y": -42.375,
+ "z": 6907.96875
+ }
+ },
+ "jump": {
+ "name": "Skaudai AO-V b47-0",
+ "coords": {
+ "x": -5340.09375,
+ "y": -520.375,
+ "z": 10196.53125
+ }
+ }
+ },
+ {
+ "title": "Heart Sector IR-V b2-0 - California Sector CQ-Y c5 (6606Ly)",
+ "prison": {
+ "name": "California Sector CQ-Y c5",
+ "coords": {
+ "x": -350.375,
+ "y": -245.53125,
+ "z": -950.625
+ }
+ },
+ "jump": {
+ "name": "Heart Sector IR-V b2-0",
+ "coords": {
+ "x": -5303.78125,
+ "y": 130.34375,
+ "z": -5305.40625
+ }
+ }
+ },
+ {
+ "title": "HIP 6024 - HR 32 (60Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 6024",
+ "coords": {
+ "x": 127.15625,
+ "y": -207.71875,
+ "z": 64.90625
+ }
+ }
+ },
+ {
+ "title": "Aspele - HR 32 (158Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Aspele",
+ "coords": {
+ "x": 117.875,
+ "y": -259.25,
+ "z": -22.1875
+ }
+ }
+ },
+ {
+ "title": "HIP 8449 - Synuefe BR-L c24-24 (157Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 8449",
+ "coords": {
+ "x": 114.3125,
+ "y": -232.59375,
+ "z": 24.34375
+ }
+ }
+ },
+ {
+ "title": "Ndano - HR 32 (152Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Ndano",
+ "coords": {
+ "x": 173.84375,
+ "y": -213.21875,
+ "z": -36.25
+ }
+ }
+ },
+ {
+ "title": "Sokaram - Col 285 Sector YU-F c11-1 (114Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Sokaram",
+ "coords": {
+ "x": 151.09375,
+ "y": -193.09375,
+ "z": 89.78125
+ }
+ }
+ },
+ {
+ "title": "Wally Berod - Col 285 Sector YU-F c11-1 (107Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Wally Berod",
+ "coords": {
+ "x": 162.0625,
+ "y": -173.8125,
+ "z": 127.9375
+ }
+ }
+ },
+ {
+ "title": "Quikuruba - Synuefe BR-L c24-24 (60Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Quikuruba",
+ "coords": {
+ "x": 188.875,
+ "y": -173.5,
+ "z": 2.03125
+ }
+ }
+ },
+ {
+ "title": "Celtici - HR 32 (87Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Celtici",
+ "coords": {
+ "x": 214.9375,
+ "y": -146.875,
+ "z": 73.1875
+ }
+ }
+ },
+ {
+ "title": "HIP 114091 - Col 285 Sector YU-F c11-1 (126Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 114091",
+ "coords": {
+ "x": 82.34375,
+ "y": -214.15625,
+ "z": 126.0
+ }
+ }
+ },
+ {
+ "title": "HIP 6369 - HR 32 (56Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 6369",
+ "coords": {
+ "x": 151.15625,
+ "y": -208.75,
+ "z": 79.75
+ }
+ }
+ },
+ {
+ "title": "NGC 6530 Sector ZE-X b2-0 - Omega Sector OD-S b4-0 (1545Ly)",
+ "prison": {
+ "name": "Omega Sector OD-S b4-0",
+ "coords": {
+ "x": -1456.8125,
+ "y": -84.8125,
+ "z": 5306.9375
+ }
+ },
+ "jump": {
+ "name": "NGC 6530 Sector ZE-X b2-0",
+ "coords": {
+ "x": -382.78125,
+ "y": -20.40625,
+ "z": 4198.09375
+ }
+ }
+ },
+ {
+ "title": "Flaming Star Sector LX-T b3-0 - California Sector CQ-Y c5 (764Ly)",
+ "prison": {
+ "name": "California Sector CQ-Y c5",
+ "coords": {
+ "x": -350.375,
+ "y": -245.53125,
+ "z": -950.625
+ }
+ },
+ "jump": {
+ "name": "Flaming Star Sector LX-T b3-0",
+ "coords": {
+ "x": -243.09375,
+ "y": -77.5625,
+ "z": -1687.71875
+ }
+ }
+ },
+ {
+ "title": "Pleiades Sector PD-S b4-0 - Pleiades Sector HR-W d1-79 (12Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Pleiades Sector PD-S b4-0",
+ "coords": {
+ "x": -80.625,
+ "y": -156.6875,
+ "z": -337.4375
+ }
+ }
+ },
+ {
+ "title": "Kaukamaye - ICZ KS-T b3-5 (112Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Kaukamaye",
+ "coords": {
+ "x": 16.1875,
+ "y": -242.53125,
+ "z": 90.4375
+ }
+ }
+ },
+ {
+ "title": "Asantani - ICZ ZK-X b1-0 (142Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "Asantani",
+ "coords": {
+ "x": 58.625,
+ "y": -244.625,
+ "z": 17.875
+ }
+ }
+ },
+ {
+ "title": "Apatugans - ICZ ZK-X b1-0 (141Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "Apatugans",
+ "coords": {
+ "x": 57.9375,
+ "y": -240.84375,
+ "z": 40.6875
+ }
+ }
+ },
+ {
+ "title": "Chhalini - ICZ KS-T b3-5 (117Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Chhalini",
+ "coords": {
+ "x": 58.9375,
+ "y": -241.25,
+ "z": 59.0
+ }
+ }
+ },
+ {
+ "title": "Jamalalam - ICZ ZK-X b1-0 (151Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "Jamalalam",
+ "coords": {
+ "x": 78.46875,
+ "y": -242.59375,
+ "z": 19.9375
+ }
+ }
+ },
+ {
+ "title": "Vocorimpus - Col 285 Sector YU-F c11-1 (132Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Vocorimpus",
+ "coords": {
+ "x": 78.09375,
+ "y": -221.0625,
+ "z": 118.75
+ }
+ }
+ },
+ {
+ "title": "Bushur - HR 32 (106Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Bushur",
+ "coords": {
+ "x": 97.9375,
+ "y": -239.8125,
+ "z": 39.15625
+ }
+ }
+ },
+ {
+ "title": "Osire Fades - Col 285 Sector YU-F c11-1 (148Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Osire Fades",
+ "coords": {
+ "x": 96.375,
+ "y": -239.0625,
+ "z": 77.5
+ }
+ }
+ },
+ {
+ "title": "Wannovik - HR 32 (73Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Wannovik",
+ "coords": {
+ "x": 99.59375,
+ "y": -222.875,
+ "z": 83.375
+ }
+ }
+ },
+ {
+ "title": "Ch'i - HR 32 (62Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Ch'i",
+ "coords": {
+ "x": 110.0625,
+ "y": -216.46875,
+ "z": 102.46875
+ }
+ }
+ },
+ {
+ "title": "Ataco - Col 285 Sector YU-F c11-1 (116Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Ataco",
+ "coords": {
+ "x": 95.625,
+ "y": -203.3125,
+ "z": 131.84375
+ }
+ }
+ },
+ {
+ "title": "Warakmbut - HR 32 (103Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Warakmbut",
+ "coords": {
+ "x": 115.03125,
+ "y": -240.4375,
+ "z": 38.46875
+ }
+ }
+ },
+ {
+ "title": "Wirnir - HR 32 (94Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Wirnir",
+ "coords": {
+ "x": 118.96875,
+ "y": -243.4375,
+ "z": 60.0625
+ }
+ }
+ },
+ {
+ "title": "Koskurni - HR 32 (110Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Koskurni",
+ "coords": {
+ "x": 120.6875,
+ "y": -220.1875,
+ "z": 8.78125
+ }
+ }
+ },
+ {
+ "title": "Njoror - HR 32 (75Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Njoror",
+ "coords": {
+ "x": 120.9375,
+ "y": -220.40625,
+ "z": 56.96875
+ }
+ }
+ },
+ {
+ "title": "Hermoricaur - Col 285 Sector YU-F c11-1 (120Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Hermoricaur",
+ "coords": {
+ "x": 133.15625,
+ "y": -207.0625,
+ "z": 107.15625
+ }
+ }
+ },
+ {
+ "title": "Wanglang Mu - Col 285 Sector YU-F c11-1 (105Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Wanglang Mu",
+ "coords": {
+ "x": 126.78125,
+ "y": -192.59375,
+ "z": 115.4375
+ }
+ }
+ },
+ {
+ "title": "Vertnyi - Synuefe BR-L c24-24 (127Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Vertnyi",
+ "coords": {
+ "x": 138.5,
+ "y": -219.90625,
+ "z": -11.875
+ }
+ }
+ },
+ {
+ "title": "Fang - Synuefe BR-L c24-24 (123Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Fang",
+ "coords": {
+ "x": 147.71875,
+ "y": -218.0,
+ "z": 21.5
+ }
+ }
+ },
+ {
+ "title": "Bugaguti - Col 285 Sector YU-F c11-1 (120Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Bugaguti",
+ "coords": {
+ "x": 150.96875,
+ "y": -200.09375,
+ "z": 103.375
+ }
+ }
+ },
+ {
+ "title": "HIP 12785 - HR 32 (89Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 12785",
+ "coords": {
+ "x": 163.375,
+ "y": -207.875,
+ "z": 31.53125
+ }
+ }
+ },
+ {
+ "title": "Yanebdjeri - Synuefe BR-L c24-24 (123Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Yanebdjeri",
+ "coords": {
+ "x": 159.875,
+ "y": -220.3125,
+ "z": 38.6875
+ }
+ }
+ },
+ {
+ "title": "Medei - Synuefe BR-L c24-24 (97Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Medei",
+ "coords": {
+ "x": 159.96875,
+ "y": -198.71875,
+ "z": -2.6875
+ }
+ }
+ },
+ {
+ "title": "Ngeratha - HR 32 (81Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Ngeratha",
+ "coords": {
+ "x": 172.78125,
+ "y": -204.8125,
+ "z": 45.84375
+ }
+ }
+ },
+ {
+ "title": "Nerremaia - HR 32 (62Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Nerremaia",
+ "coords": {
+ "x": 169.875,
+ "y": -200.375,
+ "z": 71.09375
+ }
+ }
+ },
+ {
+ "title": "Kureserians - Col 285 Sector YU-F c11-1 (126Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Kureserians",
+ "coords": {
+ "x": 159.5,
+ "y": -200.875,
+ "z": 78.53125
+ }
+ }
+ },
+ {
+ "title": "Zhuaha - HR 32 (29Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Zhuaha",
+ "coords": {
+ "x": 160.28125,
+ "y": -166.34375,
+ "z": 101.15625
+ }
+ }
+ },
+ {
+ "title": "Friguda - HR 32 (42Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Friguda",
+ "coords": {
+ "x": 174.4375,
+ "y": -156.9375,
+ "z": 100.15625
+ }
+ }
+ },
+ {
+ "title": "Puntinelao - HR 32 (63Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Puntinelao",
+ "coords": {
+ "x": 186.5625,
+ "y": -162.5,
+ "z": 67.125
+ }
+ }
+ },
+ {
+ "title": "Suluo - Bei Dou Sector DL-Y d76 (943Ly)",
+ "prison": {
+ "name": "Bei Dou Sector DL-Y d76",
+ "coords": {
+ "x": -37.6875,
+ "y": 70.09375,
+ "z": -14.1875
+ }
+ },
+ "jump": {
+ "name": "Suluo",
+ "coords": {
+ "x": 381.125,
+ "y": -384.375,
+ "z": -725.84375
+ }
+ }
+ },
+ {
+ "title": "Caister - Bei Dou Sector DL-Y d76 (937Ly)",
+ "prison": {
+ "name": "Bei Dou Sector DL-Y d76",
+ "coords": {
+ "x": -37.6875,
+ "y": 70.09375,
+ "z": -14.1875
+ }
+ },
+ "jump": {
+ "name": "Caister",
+ "coords": {
+ "x": 378.875,
+ "y": -381.4375,
+ "z": -721.875
+ }
+ }
+ },
+ {
+ "title": "HIP 4045 - ICZ KS-T b3-5 (123Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 4045",
+ "coords": {
+ "x": 50.125,
+ "y": -253.9375,
+ "z": 29.59375
+ }
+ }
+ },
+ {
+ "title": "HIP 6780 - HR 32 (107Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 6780",
+ "coords": {
+ "x": 135.96875,
+ "y": -256.5625,
+ "z": 55.96875
+ }
+ }
+ },
+ {
+ "title": "HIP 4261 - ICZ ZK-X b1-0 (152Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "HIP 4261",
+ "coords": {
+ "x": 80.8125,
+ "y": -237.53125,
+ "z": 47.78125
+ }
+ }
+ },
+ {
+ "title": "HIP 114878 - ICZ KS-T b3-5 (111Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 114878",
+ "coords": {
+ "x": -1.21875,
+ "y": -240.71875,
+ "z": 96.375
+ }
+ }
+ },
+ {
+ "title": "HIP 114333 - Col 285 Sector YU-F c11-1 (136Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 114333",
+ "coords": {
+ "x": 94.1875,
+ "y": -224.25,
+ "z": 132.8125
+ }
+ }
+ },
+ {
+ "title": "HIP 16038 - Synuefe BR-L c24-24 (131Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 16038",
+ "coords": {
+ "x": 152.46875,
+ "y": -226.59375,
+ "z": -53.46875
+ }
+ }
+ },
+ {
+ "title": "HIP 10906 - HR 32 (128Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 10906",
+ "coords": {
+ "x": 113.75,
+ "y": -228.59375,
+ "z": -6.875
+ }
+ }
+ },
+ {
+ "title": "HIP 28525 - Synuefe BR-L c24-24 (96Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 28525",
+ "coords": {
+ "x": 217.59375,
+ "y": -129.96875,
+ "z": 86.375
+ }
+ }
+ },
+ {
+ "title": "HIP 114422 - ICZ KS-T b3-5 (114Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 114422",
+ "coords": {
+ "x": 19.34375,
+ "y": -234.8125,
+ "z": 107.75
+ }
+ }
+ },
+ {
+ "title": "HIP 29314 - Synuefe BR-L c24-24 (18Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 29314",
+ "coords": {
+ "x": 220.46875,
+ "y": -118.90625,
+ "z": 2.625
+ }
+ }
+ },
+ {
+ "title": "HIP 4663 - ICZ KS-T b3-5 (122Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 4663",
+ "coords": {
+ "x": 20.3125,
+ "y": -255.84375,
+ "z": 0.71875
+ }
+ }
+ },
+ {
+ "title": "HIP 114472 - Col 285 Sector YU-F c11-1 (155Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 114472",
+ "coords": {
+ "x": 78.09375,
+ "y": -242.40625,
+ "z": 133.125
+ }
+ }
+ },
+ {
+ "title": "HIP 11585 - HR 32 (139Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 11585",
+ "coords": {
+ "x": 144.09375,
+ "y": -254.25,
+ "z": -1.53125
+ }
+ }
+ },
+ {
+ "title": "HIP 8623 - Synuefe BR-L c24-24 (142Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 8623",
+ "coords": {
+ "x": 166.0625,
+ "y": -234.40625,
+ "z": 65.46875
+ }
+ }
+ },
+ {
+ "title": "HIP 99551 - Col 285 Sector YU-F c11-1 (103Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 99551",
+ "coords": {
+ "x": 155.84375,
+ "y": -139.53125,
+ "z": 168.5625
+ }
+ }
+ },
+ {
+ "title": "Skaude ZK-X e1-203 - Nyeajaae VU-Y a27-9 (3159Ly)",
+ "prison": {
+ "name": "Nyeajaae VU-Y a27-9",
+ "coords": {
+ "x": -3374,
+ "y": -42.375,
+ "z": 6907.96875
+ }
+ },
+ "jump": {
+ "name": "Skaude ZK-X e1-203",
+ "coords": {
+ "x": -4981.15625,
+ "y": -420.65625,
+ "z": 9601.34375
+ }
+ }
+ },
+ {
+ "title": "HIP 115501 - ICZ KS-T b3-5 (108Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 115501",
+ "coords": {
+ "x": -14.375,
+ "y": -243.0,
+ "z": 83.75
+ }
+ }
+ },
+ {
+ "title": "HIP 115337 - ICZ KS-T b3-5 (132Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 115337",
+ "coords": {
+ "x": 18.8125,
+ "y": -257.4375,
+ "z": 105.3125
+ }
+ }
+ },
+ {
+ "title": "HIP 113019 - Col 285 Sector YU-F c11-1 (106Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 113019",
+ "coords": {
+ "x": 102.375,
+ "y": -192.5625,
+ "z": 131.40625
+ }
+ }
+ },
+ {
+ "title": "Ronemar - Bei Dou Sector DL-Y d76 (925Ly)",
+ "prison": {
+ "name": "Bei Dou Sector DL-Y d76",
+ "coords": {
+ "x": -37.6875,
+ "y": 70.09375,
+ "z": -14.1875
+ }
+ },
+ "jump": {
+ "name": "Ronemar",
+ "coords": {
+ "x": 355.75,
+ "y": -400.5,
+ "z": -707.21875
+ }
+ }
+ },
+ {
+ "title": "Washi - ICZ KS-T b3-5 (111Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Washi",
+ "coords": {
+ "x": 15.1875,
+ "y": -252.5,
+ "z": 42.25
+ }
+ }
+ },
+ {
+ "title": "Nehabtani - ICZ ZK-X b1-0 (146Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "Nehabtani",
+ "coords": {
+ "x": 21.34375,
+ "y": -247.375,
+ "z": 77.03125
+ }
+ }
+ },
+ {
+ "title": "Pisage - ICZ KS-T b3-5 (150Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Pisage",
+ "coords": {
+ "x": 85.4375,
+ "y": -236.6875,
+ "z": -34.46875
+ }
+ }
+ },
+ {
+ "title": "Kaleni - ICZ ZK-X b1-0 (148Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "Kaleni",
+ "coords": {
+ "x": 74.1875,
+ "y": -243.0625,
+ "z": 15.375
+ }
+ }
+ },
+ {
+ "title": "Caudji - Col 285 Sector YU-F c11-1 (151Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Caudji",
+ "coords": {
+ "x": 94.4375,
+ "y": -242.78125,
+ "z": 83.28125
+ }
+ }
+ },
+ {
+ "title": "Wallites - HR 32 (159Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Wallites",
+ "coords": {
+ "x": 111.6875,
+ "y": -259.125,
+ "z": -22.6875
+ }
+ }
+ },
+ {
+ "title": "HIP 9921 - HR 32 (112Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 9921",
+ "coords": {
+ "x": 128.28125,
+ "y": -235.4375,
+ "z": 17.125
+ }
+ }
+ },
+ {
+ "title": "Wudjari - Hyades Sector LC-L b8-0 (122Ly)",
+ "prison": {
+ "name": "Hyades Sector LC-L b8-0",
+ "coords": {
+ "x": 93.71875,
+ "y": -103.625,
+ "z": -85.9375
+ }
+ },
+ "jump": {
+ "name": "Wudjari",
+ "coords": {
+ "x": 142.5625,
+ "y": -210.4375,
+ "z": -51.34375
+ }
+ }
+ },
+ {
+ "title": "Kunapa - HR 32 (130Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Kunapa",
+ "coords": {
+ "x": 172.875,
+ "y": -218.8125,
+ "z": -8.625
+ }
+ }
+ },
+ {
+ "title": "Djerato - Synuefe BR-L c24-24 (122Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Djerato",
+ "coords": {
+ "x": 151.90625,
+ "y": -216.28125,
+ "z": 31.5
+ }
+ }
+ },
+ {
+ "title": "Sotiate - Col 285 Sector YU-F c11-1 (113Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Sotiate",
+ "coords": {
+ "x": 145.34375,
+ "y": -195.125,
+ "z": 94.53125
+ }
+ }
+ },
+ {
+ "title": "Daibo - HR 32 (35Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Daibo",
+ "coords": {
+ "x": 165.96875,
+ "y": -169.28125,
+ "z": 101.3125
+ }
+ }
+ },
+ {
+ "title": "Apis - HR 32 (55Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Apis",
+ "coords": {
+ "x": 186.125,
+ "y": -155.6875,
+ "z": 85.9375
+ }
+ }
+ },
+ {
+ "title": "HIP 11913 - Synuefe BR-L c24-24 (140Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 11913",
+ "coords": {
+ "x": 166.25,
+ "y": -252.9375,
+ "z": 16.34375
+ }
+ }
+ },
+ {
+ "title": "HIP 114638 - Col 285 Sector YU-F c11-1 (135Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 114638",
+ "coords": {
+ "x": 101.6875,
+ "y": -223.21875,
+ "z": 132.46875
+ }
+ }
+ },
+ {
+ "title": "Synuefai LX-R d5-28 - Bei Dou Sector DL-Y d76 (664Ly)",
+ "prison": {
+ "name": "Bei Dou Sector DL-Y d76",
+ "coords": {
+ "x": -37.6875,
+ "y": 70.09375,
+ "z": -14.1875
+ }
+ },
+ "jump": {
+ "name": "Synuefai LX-R d5-28",
+ "coords": {
+ "x": -244.5625,
+ "y": -218.5,
+ "z": -574.96875
+ }
+ }
+ },
+ {
+ "title": "Gliese 9086 - HR 32 (128Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Gliese 9086",
+ "coords": {
+ "x": 123.15625,
+ "y": -222.75,
+ "z": -12.0625
+ }
+ }
+ },
+ {
+ "title": "Exphiay - Puppis Sector CQ-Y b1 (265Ly)",
+ "prison": {
+ "name": "Puppis Sector CQ-Y b1",
+ "coords": {
+ "x": 53.65625,
+ "y": 3.15625,
+ "z": -46.5
+ }
+ },
+ "jump": {
+ "name": "Exphiay",
+ "coords": {
+ "x": 283.84375,
+ "y": -128.25,
+ "z": -38.9375
+ }
+ }
+ },
+ {
+ "title": "HIP 116232 - Col 285 Sector YU-F c11-1 (141Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 116232",
+ "coords": {
+ "x": 103.75,
+ "y": -231.59375,
+ "z": 122.71875
+ }
+ }
+ },
+ {
+ "title": "HIP 100539 - Col 285 Sector YU-F c11-1 (113Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 100539",
+ "coords": {
+ "x": 155.65625,
+ "y": -149.03125,
+ "z": 177.1875
+ }
+ }
+ },
+ {
+ "title": "HIP 4073 - ICZ KS-T b3-5 (128Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 4073",
+ "coords": {
+ "x": -21.71875,
+ "y": -254.46875,
+ "z": -18.09375
+ }
+ }
+ },
+ {
+ "title": "HIP 16984 - Synuefe BR-L c24-24 (111Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 16984",
+ "coords": {
+ "x": 155.0625,
+ "y": -205.125,
+ "z": -44.28125
+ }
+ }
+ },
+ {
+ "title": "HIP 20869 - HR 32 (108Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 20869",
+ "coords": {
+ "x": 211.3125,
+ "y": -172.78125,
+ "z": 27.25
+ }
+ }
+ },
+ {
+ "title": "HIP 78866 - HR 32 (80Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 78866",
+ "coords": {
+ "x": 194.84375,
+ "y": -118.9375,
+ "z": 129.375
+ }
+ }
+ },
+ {
+ "title": "HR 358 - ICZ KS-T b3-5 (125Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HR 358",
+ "coords": {
+ "x": 25.1875,
+ "y": -252.78125,
+ "z": -10.15625
+ }
+ }
+ },
+ {
+ "title": "HIP 116553 - Col 285 Sector YU-F c11-1 (138Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 116553",
+ "coords": {
+ "x": 86.0625,
+ "y": -229.78125,
+ "z": 111.0625
+ }
+ }
+ },
+ {
+ "title": "HIP 22150 - Synuefe BR-L c24-24 (54Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 22150",
+ "coords": {
+ "x": 220.875,
+ "y": -169.03125,
+ "z": 27.3125
+ }
+ }
+ },
+ {
+ "title": "HIP 14521 - Synuefe BR-L c24-24 (111Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 14521",
+ "coords": {
+ "x": 197.0625,
+ "y": -159.78125,
+ "z": 93.0
+ }
+ }
+ },
+ {
+ "title": "HIP 117114 - Col 285 Sector YU-F c11-1 (156Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 117114",
+ "coords": {
+ "x": 125.375,
+ "y": -242.8125,
+ "z": 127.9375
+ }
+ }
+ },
+ {
+ "title": "Skaudai CH-B d14-34 - Stuelou AT-J c25-24 (3893Ly)",
+ "prison": {
+ "name": "Stuelou AT-J c25-24",
+ "coords": {
+ "x": -6704.4375,
+ "y": -156.34375,
+ "z": 14101.875
+ }
+ },
+ "jump": {
+ "name": "Skaudai CH-B d14-34",
+ "coords": {
+ "x": -5481.84375,
+ "y": -579.15625,
+ "z": 10429.9375
+ }
+ }
+ },
+ {
+ "title": "HIP 117883 - ICZ KS-T b3-5 (103Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 117883",
+ "coords": {
+ "x": 14.09375,
+ "y": -242.5625,
+ "z": 64.9375
+ }
+ }
+ },
+ {
+ "title": "HIP 5796 - Col 285 Sector YU-F c11-1 (157Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 5796",
+ "coords": {
+ "x": 94.78125,
+ "y": -238.03125,
+ "z": 39.71875
+ }
+ }
+ },
+ {
+ "title": "HIP 116970 - ICZ KS-T b3-5 (112Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 116970",
+ "coords": {
+ "x": 24.84375,
+ "y": -244.375,
+ "z": 82.375
+ }
+ }
+ },
+ {
+ "title": "HIP 13159 - HR 32 (139Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 13159",
+ "coords": {
+ "x": 133.96875,
+ "y": -228.625,
+ "z": -21.0
+ }
+ }
+ },
+ {
+ "title": "HIP 117322 - Col 285 Sector YU-F c11-1 (153Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 117322",
+ "coords": {
+ "x": 98.5625,
+ "y": -244.625,
+ "z": 113.5
+ }
+ }
+ },
+ {
+ "title": "Pleiades Sector KC-V c2-4 - Pleiades Sector HR-W d1-79 (24Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Pleiades Sector KC-V c2-4",
+ "coords": {
+ "x": -88.8125,
+ "y": -148.71875,
+ "z": -321.1875
+ }
+ }
+ },
+ {
+ "title": "Eochi - ICZ KS-T b3-5 (109Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Eochi",
+ "coords": {
+ "x": 18.25,
+ "y": -250.375,
+ "z": 44.09375
+ }
+ }
+ },
+ {
+ "title": "Tai He - ICZ ZK-X b1-0 (171Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "Tai He",
+ "coords": {
+ "x": 90.21875,
+ "y": -259.90625,
+ "z": 9.09375
+ }
+ }
+ },
+ {
+ "title": "Ladakhi - HR 32 (142Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Ladakhi",
+ "coords": {
+ "x": 115.8125,
+ "y": -237.78125,
+ "z": -17.34375
+ }
+ }
+ },
+ {
+ "title": "Svarogich - HR 32 (48Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Svarogich",
+ "coords": {
+ "x": 114.34375,
+ "y": -203.78125,
+ "z": 101.53125
+ }
+ }
+ },
+ {
+ "title": "Orao - Hyades Sector LC-L b8-0 (119Ly)",
+ "prison": {
+ "name": "Hyades Sector LC-L b8-0",
+ "coords": {
+ "x": 93.71875,
+ "y": -103.625,
+ "z": -85.9375
+ }
+ },
+ "jump": {
+ "name": "Orao",
+ "coords": {
+ "x": 136.03125,
+ "y": -208.53125,
+ "z": -49.9375
+ }
+ }
+ },
+ {
+ "title": "Zacha - HR 32 (87Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Zacha",
+ "coords": {
+ "x": 139.75,
+ "y": -206.65625,
+ "z": 26.40625
+ }
+ }
+ },
+ {
+ "title": "Koeng Pora - HR 32 (56Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Koeng Pora",
+ "coords": {
+ "x": 163.5625,
+ "y": -198.375,
+ "z": 74.5625
+ }
+ }
+ },
+ {
+ "title": "Atjil - Col 285 Sector YU-F c11-1 (123Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Atjil",
+ "coords": {
+ "x": 159.9375,
+ "y": -198.28125,
+ "z": 114.84375
+ }
+ }
+ },
+ {
+ "title": "Estanatlehi - HR 32 (38Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Estanatlehi",
+ "coords": {
+ "x": 169.0625,
+ "y": -167.84375,
+ "z": 96.65625
+ }
+ }
+ },
+ {
+ "title": "Naungni - Synuefe BR-L c24-24 (62Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Naungni",
+ "coords": {
+ "x": 184.375,
+ "y": -173.1875,
+ "z": -15.3125
+ }
+ }
+ },
+ {
+ "title": "Synuefe KY-H c26-4 - Synuefe BR-L c24-24 (110Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Synuefe KY-H c26-4",
+ "coords": {
+ "x": 208.5,
+ "y": -182.5,
+ "z": 86.28125
+ }
+ }
+ },
+ {
+ "title": "Evangelis - Puppis Sector CQ-Y b1 (842Ly)",
+ "prison": {
+ "name": "Puppis Sector CQ-Y b1",
+ "coords": {
+ "x": 53.65625,
+ "y": 3.15625,
+ "z": -46.5
+ }
+ },
+ "jump": {
+ "name": "Evangelis",
+ "coords": {
+ "x": 353.75,
+ "y": -419.03125,
+ "z": -710.0
+ }
+ }
+ },
+ {
+ "title": "HIP 103854 - Col 285 Sector YU-F c11-1 (107Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 103854",
+ "coords": {
+ "x": 87.6875,
+ "y": -163.03125,
+ "z": 177.125
+ }
+ }
+ },
+ {
+ "title": "HIP 6254 - ICZ ZK-X b1-0 (164Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "HIP 6254",
+ "coords": {
+ "x": 79.5625,
+ "y": -257.71875,
+ "z": 20.125
+ }
+ }
+ },
+ {
+ "title": "HIP 117254 - ICZ KS-T b3-5 (108Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 117254",
+ "coords": {
+ "x": 27.09375,
+ "y": -240.8125,
+ "z": 78.78125
+ }
+ }
+ },
+ {
+ "title": "HIP 118106 - Col 285 Sector YU-F c11-1 (127Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 118106",
+ "coords": {
+ "x": 99.59375,
+ "y": -219.5,
+ "z": 100.46875
+ }
+ }
+ },
+ {
+ "title": "HIP 13514 - HR 32 (138Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 13514",
+ "coords": {
+ "x": 144.09375,
+ "y": -230.875,
+ "z": -17.75
+ }
+ }
+ },
+ {
+ "title": "HIP 17984 - Synuefe BR-L c24-24 (118Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 17984",
+ "coords": {
+ "x": 160.03125,
+ "y": -208.0625,
+ "z": -64.25
+ }
+ }
+ },
+ {
+ "title": "HIP 117831 - HR 32 (130Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 117831",
+ "coords": {
+ "x": 47.125,
+ "y": -256.78125,
+ "z": 85.6875
+ }
+ }
+ },
+ {
+ "title": "HIP 118228 - Col 285 Sector YU-F c11-1 (115Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 118228",
+ "coords": {
+ "x": 106.34375,
+ "y": -207.25,
+ "z": 100.28125
+ }
+ }
+ },
+ {
+ "title": "HIP 6422 - ICZ ZK-X b1-0 (160Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "HIP 6422",
+ "coords": {
+ "x": 90.78125,
+ "y": -243.65625,
+ "z": 28.4375
+ }
+ }
+ },
+ {
+ "title": "HIP 117903 - ICZ KS-T b3-5 (120Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 117903",
+ "coords": {
+ "x": 33.09375,
+ "y": -252.1875,
+ "z": 76.53125
+ }
+ }
+ },
+ {
+ "title": "HIP 102725 - HR 32 (73Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 102725",
+ "coords": {
+ "x": 115.40625,
+ "y": -150.0,
+ "z": 170.0
+ }
+ }
+ },
+ {
+ "title": "Pleiades Sector HR-W d1-41 - Pleiades Sector HR-W d1-79 (10Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Pleiades Sector HR-W d1-41",
+ "coords": {
+ "x": -83.96875,
+ "y": -146.15625,
+ "z": -334.21875
+ }
+ }
+ },
+ {
+ "title": "HIP 18702 - Hyades Sector LC-L b8-0 (102Ly)",
+ "prison": {
+ "name": "Hyades Sector LC-L b8-0",
+ "coords": {
+ "x": 93.71875,
+ "y": -103.625,
+ "z": -85.9375
+ }
+ },
+ "jump": {
+ "name": "HIP 18702",
+ "coords": {
+ "x": 146.6875,
+ "y": -190.15625,
+ "z": -78.46875
+ }
+ }
+ },
+ {
+ "title": "HIP 1672 - HR 32 (73Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 1672",
+ "coords": {
+ "x": 142.34375,
+ "y": -230.8125,
+ "z": 110.375
+ }
+ }
+ },
+ {
+ "title": "Boelts YK-P c21-5 - Stuelou AT-J c25-24 (2623Ly)",
+ "prison": {
+ "name": "Stuelou AT-J c25-24",
+ "coords": {
+ "x": -6704.4375,
+ "y": -156.34375,
+ "z": 14101.875
+ }
+ },
+ "jump": {
+ "name": "Boelts YK-P c21-5",
+ "coords": {
+ "x": -7709.53125,
+ "y": -454.8125,
+ "z": 16506.375
+ }
+ }
+ },
+ {
+ "title": "Pleiades Sector HR-W d1-42 - Pleiades Sector HR-W d1-79 (11Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Pleiades Sector HR-W d1-42",
+ "coords": {
+ "x": -70.1875,
+ "y": -150.21875,
+ "z": -343.84375
+ }
+ }
+ },
+ {
+ "title": "HIP 14415 - Synuefe BR-L c24-24 (120Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 14415",
+ "coords": {
+ "x": 139.59375,
+ "y": -209.75,
+ "z": -21.1875
+ }
+ }
+ },
+ {
+ "title": "Synuefai EB-R c7-5 - Bei Dou Sector DL-Y d76 (816Ly)",
+ "prison": {
+ "name": "Bei Dou Sector DL-Y d76",
+ "coords": {
+ "x": -37.6875,
+ "y": 70.09375,
+ "z": -14.1875
+ }
+ },
+ "jump": {
+ "name": "Synuefai EB-R c7-5",
+ "coords": {
+ "x": -291.59375,
+ "y": -224.375,
+ "z": -731.09375
+ }
+ }
+ },
+ {
+ "title": "Smojue PZ-R c4-5 - Omega Sector OD-S b4-0 (2666Ly)",
+ "prison": {
+ "name": "Omega Sector OD-S b4-0",
+ "coords": {
+ "x": -1456.8125,
+ "y": -84.8125,
+ "z": 5306.9375
+ }
+ },
+ "jump": {
+ "name": "Smojue PZ-R c4-5",
+ "coords": {
+ "x": -149.5625,
+ "y": 155.9375,
+ "z": 2995.625
+ }
+ }
+ },
+ {
+ "title": "Pleiades Sector IH-V c2-5 - Pleiades Sector HR-W d1-79 (23Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Pleiades Sector IH-V c2-5",
+ "coords": {
+ "x": -78.71875,
+ "y": -138.03125,
+ "z": -321.78125
+ }
+ }
+ },
+ {
+ "title": "Karm - ICZ KS-T b3-5 (106Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Karm",
+ "coords": {
+ "x": 16.03125,
+ "y": -247.8125,
+ "z": 47.84375
+ }
+ }
+ },
+ {
+ "title": "Kilique - HR 32 (146Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Kilique",
+ "coords": {
+ "x": 90.1875,
+ "y": -264.375,
+ "z": 7.0
+ }
+ }
+ },
+ {
+ "title": "Mechehooit - Col 285 Sector YU-F c11-1 (148Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Mechehooit",
+ "coords": {
+ "x": 87.59375,
+ "y": -232.0,
+ "z": 50.09375
+ }
+ }
+ },
+ {
+ "title": "Kwiambe - ICZ ZK-X b1-0 (168Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "Kwiambe",
+ "coords": {
+ "x": 98.25,
+ "y": -249.21875,
+ "z": 19.53125
+ }
+ }
+ },
+ {
+ "title": "Wonga - Col 285 Sector YU-F c11-1 (156Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Wonga",
+ "coords": {
+ "x": 113.0,
+ "y": -247.4375,
+ "z": 89.8125
+ }
+ }
+ },
+ {
+ "title": "Ogdoad - Synuefe BR-L c24-24 (119Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Ogdoad",
+ "coords": {
+ "x": 139.90625,
+ "y": -204.375,
+ "z": -36.875
+ }
+ }
+ },
+ {
+ "title": "Atunung - Synuefe BR-L c24-24 (110Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Atunung",
+ "coords": {
+ "x": 150.75,
+ "y": -207.34375,
+ "z": 5.4375
+ }
+ }
+ },
+ {
+ "title": "Bijemayah - Synuefe BR-L c24-24 (122Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Bijemayah",
+ "coords": {
+ "x": 140.90625,
+ "y": -208.75,
+ "z": 25.3125
+ }
+ }
+ },
+ {
+ "title": "Sandjin - Synuefe BR-L c24-24 (144Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Sandjin",
+ "coords": {
+ "x": 158.25,
+ "y": -224.875,
+ "z": 74.0
+ }
+ }
+ },
+ {
+ "title": "HR 1364 - HR 32 (167Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HR 1364",
+ "coords": {
+ "x": 166.71875,
+ "y": -178.375,
+ "z": -62.65625
+ }
+ }
+ },
+ {
+ "title": "Ipison - Bei Dou Sector DL-Y d76 (946Ly)",
+ "prison": {
+ "name": "Bei Dou Sector DL-Y d76",
+ "coords": {
+ "x": -37.6875,
+ "y": 70.09375,
+ "z": -14.1875
+ }
+ },
+ "jump": {
+ "name": "Ipison",
+ "coords": {
+ "x": 389.4375,
+ "y": -383.375,
+ "z": -726.28125
+ }
+ }
+ },
+ {
+ "title": "Jellyfish Sector FB-X c1-5 - California Sector CQ-Y c5 (4185Ly)",
+ "prison": {
+ "name": "California Sector CQ-Y c5",
+ "coords": {
+ "x": -350.375,
+ "y": -245.53125,
+ "z": -950.625
+ }
+ },
+ "jump": {
+ "name": "Jellyfish Sector FB-X c1-5",
+ "coords": {
+ "x": 788.40625,
+ "y": 255.46875,
+ "z": -4946.4375
+ }
+ }
+ },
+ {
+ "title": "Thor's Helmet Sector FB-X c1-5 - HR 3005 (3084Ly)",
+ "prison": {
+ "name": "HR 3005",
+ "coords": {
+ "x": 750.53125,
+ "y": -169.65625,
+ "z": -90
+ }
+ },
+ "jump": {
+ "name": "Thor's Helmet Sector FB-X c1-5",
+ "coords": {
+ "x": 2704.96875,
+ "y": -23.25,
+ "z": -2470.78125
+ }
+ }
+ },
+ {
+ "title": "HIP 19213 - HR 32 (162Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 19213",
+ "coords": {
+ "x": 172.1875,
+ "y": -193.1875,
+ "z": -53.84375
+ }
+ }
+ },
+ {
+ "title": "Rudrusa - Synuefe BR-L c24-24 (34Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Rudrusa",
+ "coords": {
+ "x": 212.34375,
+ "y": -137.4375,
+ "z": 19.9375
+ }
+ }
+ },
+ {
+ "title": "HIP 2881 - HR 32 (65Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 2881",
+ "coords": {
+ "x": 120.15625,
+ "y": -221.84375,
+ "z": 86.40625
+ }
+ }
+ },
+ {
+ "title": "Hamlet's Harmony - Eol Prou LW-L c8-127 (25Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Hamlet's Harmony",
+ "coords": {
+ "x": -9549.4375,
+ "y": -909.1875,
+ "z": 19795.875
+ }
+ }
+ },
+ {
+ "title": "HIP 116528 - ICZ KS-T b3-5 (118Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 116528",
+ "coords": {
+ "x": 4.59375,
+ "y": -254.46875,
+ "z": 81.90625
+ }
+ }
+ },
+ {
+ "title": "Gliese 67.2 - ICZ ZK-X b1-0 (158Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "Gliese 67.2",
+ "coords": {
+ "x": 91.34375,
+ "y": -241.5625,
+ "z": 10.125
+ }
+ }
+ },
+ {
+ "title": "HIP 104065 - Col 285 Sector YU-F c11-1 (112Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 104065",
+ "coords": {
+ "x": 111.53125,
+ "y": -167.875,
+ "z": 180.0
+ }
+ }
+ },
+ {
+ "title": "Juniper - Eol Prou LW-L c8-127 (29Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Juniper",
+ "coords": {
+ "x": -9557.9375,
+ "y": -911.59375,
+ "z": 19806.0625
+ }
+ }
+ },
+ {
+ "title": "Loptanii - ICZ KS-T b3-5 (123Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Loptanii",
+ "coords": {
+ "x": 7.59375,
+ "y": -259.84375,
+ "z": 80.25
+ }
+ }
+ },
+ {
+ "title": "HIP 14836 - Synuefe BR-L c24-24 (108Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 14836",
+ "coords": {
+ "x": 155.40625,
+ "y": -209.1875,
+ "z": -8.40625
+ }
+ }
+ },
+ {
+ "title": "HIP 116647 - Col 285 Sector YU-F c11-1 (92Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 116647",
+ "coords": {
+ "x": 138.0,
+ "y": -173.1875,
+ "z": 116.6875
+ }
+ }
+ },
+ {
+ "title": "HIP 3864 - HR 32 (96Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 3864",
+ "coords": {
+ "x": 104.0625,
+ "y": -245.5625,
+ "z": 67.09375
+ }
+ }
+ },
+ {
+ "title": "HIP 14361 - Hyades Sector LC-L b8-0 (131Ly)",
+ "prison": {
+ "name": "Hyades Sector LC-L b8-0",
+ "coords": {
+ "x": 93.71875,
+ "y": -103.625,
+ "z": -85.9375
+ }
+ },
+ "jump": {
+ "name": "HIP 14361",
+ "coords": {
+ "x": 125.96875,
+ "y": -226.15625,
+ "z": -51.28125
+ }
+ }
+ },
+ {
+ "title": "White Sun - Eol Prou LW-L c8-127 (45Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "White Sun",
+ "coords": {
+ "x": -9521.0,
+ "y": -916.125,
+ "z": 19853.1875
+ }
+ }
+ },
+ {
+ "title": "Keiadiras - ICZ KS-T b3-5 (130Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Keiadiras",
+ "coords": {
+ "x": 13.75,
+ "y": -258.5625,
+ "z": 99.4375
+ }
+ }
+ },
+ {
+ "title": "HIP 112549 - HR 32 (88Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 112549",
+ "coords": {
+ "x": 63.28125,
+ "y": -205.0625,
+ "z": 128.5
+ }
+ }
+ },
+ {
+ "title": "HIP 106615 - Col 285 Sector YU-F c11-1 (120Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 106615",
+ "coords": {
+ "x": 81.71875,
+ "y": -184.5,
+ "z": 172.34375
+ }
+ }
+ },
+ {
+ "title": "HIP 4273 - HR 32 (68Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 4273",
+ "coords": {
+ "x": 149.53125,
+ "y": -225.25,
+ "z": 93.59375
+ }
+ }
+ },
+ {
+ "title": "Kioti 368 - Eol Prou LW-L c8-127 (33Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Kioti 368",
+ "coords": {
+ "x": -9531.125,
+ "y": -910.4375,
+ "z": 19776.1875
+ }
+ }
+ },
+ {
+ "title": "Guinasan - ICZ KS-T b3-5 (129Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Guinasan",
+ "coords": {
+ "x": -39.84375,
+ "y": -253.625,
+ "z": 98.6875
+ }
+ }
+ },
+ {
+ "title": "HIP 10413 - HR 32 (145Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 10413",
+ "coords": {
+ "x": 94.125,
+ "y": -232.125,
+ "z": -20.09375
+ }
+ }
+ },
+ {
+ "title": "HIP 113921 - Col 285 Sector YU-F c11-1 (130Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 113921",
+ "coords": {
+ "x": 71.0625,
+ "y": -216.53125,
+ "z": 124.53125
+ }
+ }
+ },
+ {
+ "title": "HIP 9408 - HR 32 (144Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 9408",
+ "coords": {
+ "x": 100.78125,
+ "y": -251.375,
+ "z": -6.46875
+ }
+ }
+ },
+ {
+ "title": "HIP 106311 - Col 285 Sector YU-F c11-1 (94Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 106311",
+ "coords": {
+ "x": 143.3125,
+ "y": -154.09375,
+ "z": 152.84375
+ }
+ }
+ },
+ {
+ "title": "Forsina - Col 285 Sector YU-F c11-1 (104Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Forsina",
+ "coords": {
+ "x": 187.34375,
+ "y": -146.15625,
+ "z": 97.53125
+ }
+ }
+ },
+ {
+ "title": "Col 359 Sector UM-T c4-6 - Alrai Sector KN-S b4-4 (230Ly)",
+ "prison": {
+ "name": "Alrai Sector KN-S b4-4",
+ "coords": {
+ "x": -34.96875,
+ "y": 77.625,
+ "z": 101.59375
+ }
+ },
+ "jump": {
+ "name": "Col 359 Sector UM-T c4-6",
+ "coords": {
+ "x": -4.96875,
+ "y": 64.46875,
+ "z": 329.28125
+ }
+ }
+ },
+ {
+ "title": "Saelishi - ICZ KS-T b3-5 (139Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Saelishi",
+ "coords": {
+ "x": 51.8125,
+ "y": -257.34375,
+ "z": -15.0
+ }
+ }
+ },
+ {
+ "title": "Muan - ICZ KS-T b3-5 (158Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Muan",
+ "coords": {
+ "x": 89.03125,
+ "y": -236.8125,
+ "z": -44.90625
+ }
+ }
+ },
+ {
+ "title": "Giras - HR 32 (146Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Giras",
+ "coords": {
+ "x": 92.78125,
+ "y": -262.40625,
+ "z": 3.84375
+ }
+ }
+ },
+ {
+ "title": "Ankarawai - HR 32 (99Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Ankarawai",
+ "coords": {
+ "x": 91.875,
+ "y": -241.71875,
+ "z": 63.125
+ }
+ }
+ },
+ {
+ "title": "Kuwemaki - Synuefe BR-L c24-24 (135Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Kuwemaki",
+ "coords": {
+ "x": 134.65625,
+ "y": -226.90625,
+ "z": -7.8125
+ }
+ }
+ },
+ {
+ "title": "Konoto - HR 32 (121Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Konoto",
+ "coords": {
+ "x": 96.90625,
+ "y": -245.90625,
+ "z": 22.21875
+ }
+ }
+ },
+ {
+ "title": "Bhumian - Col 285 Sector YU-F c11-1 (158Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Bhumian",
+ "coords": {
+ "x": 108.65625,
+ "y": -250.71875,
+ "z": 93.28125
+ }
+ }
+ },
+ {
+ "title": "Hania - HR 32 (74Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Hania",
+ "coords": {
+ "x": 105.25,
+ "y": -222.59375,
+ "z": 71.46875
+ }
+ }
+ },
+ {
+ "title": "Wen - Hyades Sector LC-L b8-0 (112Ly)",
+ "prison": {
+ "name": "Hyades Sector LC-L b8-0",
+ "coords": {
+ "x": 93.71875,
+ "y": -103.625,
+ "z": -85.9375
+ }
+ },
+ "jump": {
+ "name": "Wen",
+ "coords": {
+ "x": 139.125,
+ "y": -202.90625,
+ "z": -60.40625
+ }
+ }
+ },
+ {
+ "title": "Aurvasude - HR 32 (92Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Aurvasude",
+ "coords": {
+ "x": 143.0625,
+ "y": -211.3125,
+ "z": 23.78125
+ }
+ }
+ },
+ {
+ "title": "Paentun - Col 285 Sector YU-F c11-1 (117Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Paentun",
+ "coords": {
+ "x": 154.71875,
+ "y": -192.125,
+ "z": 70.40625
+ }
+ }
+ },
+ {
+ "title": "Kumal - Col 285 Sector YU-F c11-1 (114Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Kumal",
+ "coords": {
+ "x": 161.03125,
+ "y": -188.15625,
+ "z": 100.0625
+ }
+ }
+ },
+ {
+ "title": "Sumeragwa - Synuefe BR-L c24-24 (87Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Sumeragwa",
+ "coords": {
+ "x": 197.9375,
+ "y": -146.59375,
+ "z": 70.46875
+ }
+ }
+ },
+ {
+ "title": "HIP 11469 - Synuefe BR-L c24-24 (132Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 11469",
+ "coords": {
+ "x": 166.34375,
+ "y": -239.28125,
+ "z": 30.84375
+ }
+ }
+ },
+ {
+ "title": "HIP 4934 - HR 32 (68Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 4934",
+ "coords": {
+ "x": 123.21875,
+ "y": -220.25,
+ "z": 70.28125
+ }
+ }
+ },
+ {
+ "title": "Trifid Sector IR-W d1-52 - Omega Sector OD-S b4-0 (855Ly)",
+ "prison": {
+ "name": "Omega Sector OD-S b4-0",
+ "coords": {
+ "x": -1456.8125,
+ "y": -84.8125,
+ "z": 5306.9375
+ }
+ },
+ "jump": {
+ "name": "Trifid Sector IR-W d1-52",
+ "coords": {
+ "x": -612.40625,
+ "y": -31.5,
+ "z": 5182.875
+ }
+ }
+ },
+ {
+ "title": "HIP 1187 - ICZ ZK-X b1-0 (129Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "HIP 1187",
+ "coords": {
+ "x": -11.0,
+ "y": -248.84375,
+ "z": 31.75
+ }
+ }
+ },
+ {
+ "title": "HIP 118079 - Col 285 Sector YU-F c11-1 (99Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 118079",
+ "coords": {
+ "x": 150.15625,
+ "y": -174.75,
+ "z": 116.1875
+ }
+ }
+ },
+ {
+ "title": "HIP 18496 - HR 32 (165Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 18496",
+ "coords": {
+ "x": 161.375,
+ "y": -197.5,
+ "z": -58.9375
+ }
+ }
+ },
+ {
+ "title": "HIP 13341 - HR 32 (115Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 13341",
+ "coords": {
+ "x": 143.25,
+ "y": -212.90625,
+ "z": -1.875
+ }
+ }
+ },
+ {
+ "title": "HIP 6182 - ICZ KS-T b3-5 (137Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 6182",
+ "coords": {
+ "x": 43.96875,
+ "y": -262.09375,
+ "z": -5.90625
+ }
+ }
+ },
+ {
+ "title": "HIP 18563 - HR 32 (173Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "HIP 18563",
+ "coords": {
+ "x": 156.0,
+ "y": -196.25,
+ "z": -68.5
+ }
+ }
+ },
+ {
+ "title": "HIP 13979 - Synuefe BR-L c24-24 (121Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 13979",
+ "coords": {
+ "x": 136.5625,
+ "y": -208.90625,
+ "z": -16.3125
+ }
+ }
+ },
+ {
+ "title": "HIP 110105 - Col 285 Sector YU-F c11-1 (108Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 110105",
+ "coords": {
+ "x": 140.0625,
+ "y": -177.0,
+ "z": 151.03125
+ }
+ }
+ },
+ {
+ "title": "Synuefe BT-F d12-54 - Synuefe BR-L c24-24 (17Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Synuefe BT-F d12-54",
+ "coords": {
+ "x": 228.875,
+ "y": -117.125,
+ "z": 1.6875
+ }
+ }
+ },
+ {
+ "title": "Delphi - Pleiades Sector HR-W d1-79 (30Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Delphi",
+ "coords": {
+ "x": -63.59375,
+ "y": -147.40625,
+ "z": -319.09375
+ }
+ }
+ },
+ {
+ "title": "HIP 14045 - Synuefe BR-L c24-24 (120Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "HIP 14045",
+ "coords": {
+ "x": 139.96875,
+ "y": -211.65625,
+ "z": -15.6875
+ }
+ }
+ },
+ {
+ "title": "Nai No Kami - Col 285 Sector YU-F c11-1 (156Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Nai No Kami",
+ "coords": {
+ "x": 133.125,
+ "y": -244.46875,
+ "z": 110.84375
+ }
+ }
+ },
+ {
+ "title": "HIP 111096 - Col 285 Sector YU-F c11-1 (95Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 111096",
+ "coords": {
+ "x": 151.125,
+ "y": -160.5,
+ "z": 138.8125
+ }
+ }
+ },
+ {
+ "title": "HIP 116263 - Col 285 Sector YU-F c11-1 (143Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 116263",
+ "coords": {
+ "x": 91.59375,
+ "y": -234.09375,
+ "z": 117.78125
+ }
+ }
+ },
+ {
+ "title": "Chongloi - Synuefe BR-L c24-24 (134Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Chongloi",
+ "coords": {
+ "x": 169.46875,
+ "y": -213.28125,
+ "z": 77.59375
+ }
+ }
+ },
+ {
+ "title": "Sokathani - Synuefe BR-L c24-24 (48Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Sokathani",
+ "coords": {
+ "x": 200.0625,
+ "y": -168.46875,
+ "z": -14.78125
+ }
+ }
+ },
+ {
+ "title": "Pleiades Sector HR-W d1-57 - Pleiades Sector HR-W d1-79 (47Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Pleiades Sector HR-W d1-57",
+ "coords": {
+ "x": -86.40625,
+ "y": -125.15625,
+ "z": -301.84375
+ }
+ }
+ },
+ {
+ "title": "HIP 6761 - ICZ KS-T b3-5 (142Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 6761",
+ "coords": {
+ "x": 35.6875,
+ "y": -263.625,
+ "z": -21.84375
+ }
+ }
+ },
+ {
+ "title": "HIP 116663 - Col 285 Sector YU-F c11-1 (175Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 116663",
+ "coords": {
+ "x": 56.96875,
+ "y": -262.59375,
+ "z": 106.8125
+ }
+ }
+ },
+ {
+ "title": "Guinna - HR 32 (96Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Guinna",
+ "coords": {
+ "x": 216.71875,
+ "y": -131.0,
+ "z": 61.78125
+ }
+ }
+ },
+ {
+ "title": "HIP 8722 - ICZ KS-T b3-5 (151Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "HIP 8722",
+ "coords": {
+ "x": 81.4375,
+ "y": -255.1875,
+ "z": -14.15625
+ }
+ }
+ },
+ {
+ "title": "Theta Phoenicis - ICZ KS-T b3-5 (115Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Theta Phoenicis",
+ "coords": {
+ "x": 45.4375,
+ "y": -235.71875,
+ "z": 92.40625
+ }
+ }
+ },
+ {
+ "title": "California Sector BV-Y c7 - Bei Dou Sector DL-Y d76 (1028Ly)",
+ "prison": {
+ "name": "Bei Dou Sector DL-Y d76",
+ "coords": {
+ "x": -37.6875,
+ "y": 70.09375,
+ "z": -14.1875
+ }
+ },
+ "jump": {
+ "name": "California Sector BV-Y c7",
+ "coords": {
+ "x": -342.3125,
+ "y": -219.28125,
+ "z": -952.71875
+ }
+ }
+ },
+ {
+ "title": "Pleiades Sector IH-V c2-7 - Pleiades Sector HR-W d1-79 (19Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Pleiades Sector IH-V c2-7",
+ "coords": {
+ "x": -78.46875,
+ "y": -144.9375,
+ "z": -324.9375
+ }
+ }
+ },
+ {
+ "title": "Kondareni - ICZ KS-T b3-5 (135Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Kondareni",
+ "coords": {
+ "x": 24.4375,
+ "y": -259.71875,
+ "z": -19.875
+ }
+ }
+ },
+ {
+ "title": "Arug - ICZ KS-T b3-5 (116Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Arug",
+ "coords": {
+ "x": 30.53125,
+ "y": -253.875,
+ "z": 52.65625
+ }
+ }
+ },
+ {
+ "title": "Veroklist - ICZ KS-T b3-5 (131Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Veroklist",
+ "coords": {
+ "x": 47.3125,
+ "y": -260.09375,
+ "z": 73.5625
+ }
+ }
+ },
+ {
+ "title": "Any Nal - ICZ KS-T b3-5 (155Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Any Nal",
+ "coords": {
+ "x": 85.34375,
+ "y": -232.875,
+ "z": -47.4375
+ }
+ }
+ },
+ {
+ "title": "Thakan - HR 32 (146Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Thakan",
+ "coords": {
+ "x": 134.0,
+ "y": -242.28125,
+ "z": -20.71875
+ }
+ }
+ },
+ {
+ "title": "Heimanes - ICZ ZK-X b1-0 (169Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "Heimanes",
+ "coords": {
+ "x": 97.78125,
+ "y": -250.375,
+ "z": 23.40625
+ }
+ }
+ },
+ {
+ "title": "Masar - HR 32 (90Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Masar",
+ "coords": {
+ "x": 133.6875,
+ "y": -248.53125,
+ "z": 86.53125
+ }
+ }
+ },
+ {
+ "title": "Wuninka - Col 285 Sector YU-F c11-1 (129Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Wuninka",
+ "coords": {
+ "x": 120.84375,
+ "y": -218.625,
+ "z": 117.3125
+ }
+ }
+ },
+ {
+ "title": "Bhagiriyot - Hyades Sector LC-L b8-0 (122Ly)",
+ "prison": {
+ "name": "Hyades Sector LC-L b8-0",
+ "coords": {
+ "x": 93.71875,
+ "y": -103.625,
+ "z": -85.9375
+ }
+ },
+ "jump": {
+ "name": "Bhagiriyot",
+ "coords": {
+ "x": 144.84375,
+ "y": -210.75,
+ "z": -57.875
+ }
+ }
+ },
+ {
+ "title": "Privisci - Synuefe BR-L c24-24 (112Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Privisci",
+ "coords": {
+ "x": 153.1875,
+ "y": -212.0,
+ "z": 4.65625
+ }
+ }
+ },
+ {
+ "title": "Ragana - Synuefe BR-L c24-24 (125Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Ragana",
+ "coords": {
+ "x": 142.875,
+ "y": -208.4375,
+ "z": 38.78125
+ }
+ }
+ },
+ {
+ "title": "Koing - Synuefe BR-L c24-24 (135Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Koing",
+ "coords": {
+ "x": 156.6875,
+ "y": -220.59375,
+ "z": 60.40625
+ }
+ }
+ },
+ {
+ "title": "Grud - Synuefe BR-L c24-24 (86Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Grud",
+ "coords": {
+ "x": 191.75,
+ "y": -149.4375,
+ "z": 66.8125
+ }
+ }
+ },
+ {
+ "title": "Lembass - Bei Dou Sector DL-Y d76 (956Ly)",
+ "prison": {
+ "name": "Bei Dou Sector DL-Y d76",
+ "coords": {
+ "x": -37.6875,
+ "y": 70.09375,
+ "z": -14.1875
+ }
+ },
+ "jump": {
+ "name": "Lembass",
+ "coords": {
+ "x": 385.625,
+ "y": -388.21875,
+ "z": -738.09375
+ }
+ }
+ },
+ {
+ "title": "Wisanye - Bei Dou Sector DL-Y d76 (936Ly)",
+ "prison": {
+ "name": "Bei Dou Sector DL-Y d76",
+ "coords": {
+ "x": -37.6875,
+ "y": 70.09375,
+ "z": -14.1875
+ }
+ },
+ "jump": {
+ "name": "Wisanye",
+ "coords": {
+ "x": 387.40625,
+ "y": -383.71875,
+ "z": -714.34375
+ }
+ }
+ },
+ {
+ "title": "Rosette Sector CQ-Y d59 - California Sector CQ-Y c5 (4662Ly)",
+ "prison": {
+ "name": "California Sector CQ-Y c5",
+ "coords": {
+ "x": -350.375,
+ "y": -245.53125,
+ "z": -950.625
+ }
+ },
+ "jump": {
+ "name": "Rosette Sector CQ-Y d59",
+ "coords": {
+ "x": 2345.96875,
+ "y": -167.4375,
+ "z": -4752.90625
+ }
+ }
+ },
+ {
+ "title": "Barangali - HR 32 (75Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Barangali",
+ "coords": {
+ "x": 134.71875,
+ "y": -230.40625,
+ "z": 76.75
+ }
+ }
+ },
+ {
+ "title": "Lysoosms YS-U d2-61 - Nyeajaae VU-Y a27-9 (447Ly)",
+ "prison": {
+ "name": "Nyeajaae VU-Y a27-9",
+ "coords": {
+ "x": -3374,
+ "y": -42.375,
+ "z": 6907.96875
+ }
+ },
+ "jump": {
+ "name": "Lysoosms YS-U d2-61",
+ "coords": {
+ "x": -2931.46875,
+ "y": 3.46875,
+ "z": 6867.625
+ }
+ }
+ },
+ {
+ "title": "Yamari - ICZ KS-T b3-5 (150Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Yamari",
+ "coords": {
+ "x": 82.15625,
+ "y": -258.75,
+ "z": -1.4375
+ }
+ }
+ },
+ {
+ "title": "Koenpeiadal - Synuefe BR-L c24-24 (137Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Koenpeiadal",
+ "coords": {
+ "x": 153.59375,
+ "y": -243.5625,
+ "z": 7.28125
+ }
+ }
+ },
+ {
+ "title": "Kokondji - HR 32 (97Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Kokondji",
+ "coords": {
+ "x": 161.15625,
+ "y": -242.625,
+ "z": 59.28125
+ }
+ }
+ },
+ {
+ "title": "Pleiades Sector JC-V d2-62 - Pleiades Sector HR-W d1-79 (94Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Pleiades Sector JC-V d2-62",
+ "coords": {
+ "x": -82.1875,
+ "y": -100.9375,
+ "z": -260.59375
+ }
+ }
+ },
+ {
+ "title": "Ibisanyan - HR 32 (131Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Ibisanyan",
+ "coords": {
+ "x": 150.65625,
+ "y": -236.4375,
+ "z": -5.28125
+ }
+ }
+ },
+ {
+ "title": "Pleiades Sector DL-Y d65 - Pleiades Sector HR-W d1-79 (7Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Pleiades Sector DL-Y d65",
+ "coords": {
+ "x": -83.28125,
+ "y": -151.40625,
+ "z": -347.1875
+ }
+ }
+ },
+ {
+ "title": "Moku - HR 32 (81Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Moku",
+ "coords": {
+ "x": 162.0625,
+ "y": -193.15625,
+ "z": 32.125
+ }
+ }
+ },
+ {
+ "title": "Bahilo - Col 285 Sector YU-F c11-1 (139Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Bahilo",
+ "coords": {
+ "x": 131.9375,
+ "y": -223.25,
+ "z": 65.53125
+ }
+ }
+ },
+ {
+ "title": "Crescent Sector GW-W c1-8 - Capricorni Sector FM-V b2-0 (4887Ly)",
+ "prison": {
+ "name": "Capricorni Sector FM-V b2-0",
+ "coords": {
+ "x": -103.59375,
+ "y": -100.375,
+ "z": 97.4375
+ }
+ },
+ "jump": {
+ "name": "Crescent Sector GW-W c1-8",
+ "coords": {
+ "x": -4842.1875,
+ "y": 210.8125,
+ "z": 1252.21875
+ }
+ }
+ },
+ {
+ "title": "Ceos - G 203-51 (479Ly)",
+ "prison": {
+ "name": "G 203-51",
+ "coords": {
+ "x": -15.875,
+ "y": 12.03125,
+ "z": 5.34375
+ }
+ },
+ "jump": {
+ "name": "Ceos",
+ "coords": {
+ "x": -348.65625,
+ "y": 13.875,
+ "z": -339.21875
+ }
+ }
+ },
+ {
+ "title": "Ahaut - ICZ ZK-X b1-0 (149Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "Ahaut",
+ "coords": {
+ "x": 51.21875,
+ "y": -246.125,
+ "z": -42.15625
+ }
+ }
+ },
+ {
+ "title": "Nagni - ICZ ZK-X b1-0 (163Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "Nagni",
+ "coords": {
+ "x": 85.21875,
+ "y": -253.0,
+ "z": 15.46875
+ }
+ }
+ },
+ {
+ "title": "Ngolock - HR 32 (84Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Ngolock",
+ "coords": {
+ "x": 141.65625,
+ "y": -233.65625,
+ "z": 61.125
+ }
+ }
+ },
+ {
+ "title": "HIP 104206 - Col 285 Sector YU-F c11-1 (91Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 104206",
+ "coords": {
+ "x": 161.0,
+ "y": -137.5,
+ "z": 145.375
+ }
+ }
+ },
+ {
+ "title": "Mel 22 Sector GM-V c2-8 - Bei Dou Sector DL-Y d76 (551Ly)",
+ "prison": {
+ "name": "Bei Dou Sector DL-Y d76",
+ "coords": {
+ "x": -37.6875,
+ "y": 70.09375,
+ "z": -14.1875
+ }
+ },
+ "jump": {
+ "name": "Mel 22 Sector GM-V c2-8",
+ "coords": {
+ "x": -192.125,
+ "y": -194.96875,
+ "z": -471.65625
+ }
+ }
+ },
+ {
+ "title": "Annarthia - ICZ KS-T b3-5 (118Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Annarthia",
+ "coords": {
+ "x": 41.5,
+ "y": -252.25,
+ "z": 37.625
+ }
+ }
+ },
+ {
+ "title": "Inherdis - ICZ KS-T b3-5 (127Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Inherdis",
+ "coords": {
+ "x": 49.15625,
+ "y": -255.3125,
+ "z": 72.4375
+ }
+ }
+ },
+ {
+ "title": "Gerdulla - ICZ ZK-X b1-0 (161Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "Gerdulla",
+ "coords": {
+ "x": 63.75,
+ "y": -263.625,
+ "z": 8.125
+ }
+ }
+ },
+ {
+ "title": "Yarraluk - HR 32 (147Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Yarraluk",
+ "coords": {
+ "x": 131.15625,
+ "y": -240.9375,
+ "z": -23.125
+ }
+ }
+ },
+ {
+ "title": "Aman Zaihe - HR 32 (129Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Aman Zaihe",
+ "coords": {
+ "x": 105.9375,
+ "y": -255.9375,
+ "z": 17.28125
+ }
+ }
+ },
+ {
+ "title": "Narim - Hyades Sector LC-L b8-0 (119Ly)",
+ "prison": {
+ "name": "Hyades Sector LC-L b8-0",
+ "coords": {
+ "x": 93.71875,
+ "y": -103.625,
+ "z": -85.9375
+ }
+ },
+ "jump": {
+ "name": "Narim",
+ "coords": {
+ "x": 142.65625,
+ "y": -207.4375,
+ "z": -54.25
+ }
+ }
+ },
+ {
+ "title": "Grealli - Synuefe BR-L c24-24 (108Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Grealli",
+ "coords": {
+ "x": 174.9375,
+ "y": -220.1875,
+ "z": 16.59375
+ }
+ }
+ },
+ {
+ "title": "Anumanas - Synuefe BR-L c24-24 (138Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Anumanas",
+ "coords": {
+ "x": 150.59375,
+ "y": -223.09375,
+ "z": 56.9375
+ }
+ }
+ },
+ {
+ "title": "Ngalakan - HR 32 (28Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Ngalakan",
+ "coords": {
+ "x": 154.25,
+ "y": -176.34375,
+ "z": 97.625
+ }
+ }
+ },
+ {
+ "title": "Dalat - Synuefe BR-L c24-24 (45Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Dalat",
+ "coords": {
+ "x": 196.65625,
+ "y": -159.15625,
+ "z": 4.71875
+ }
+ }
+ },
+ {
+ "title": "Guugupala - HR 32 (96Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Guugupala",
+ "coords": {
+ "x": 185.5,
+ "y": -178.21875,
+ "z": 20.8125
+ }
+ }
+ },
+ {
+ "title": "Edensu - Synuefe BR-L c24-24 (104Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Edensu",
+ "coords": {
+ "x": 205.65625,
+ "y": -166.28125,
+ "z": 86.25
+ }
+ }
+ },
+ {
+ "title": "Eravins - ICZ ZK-X b1-0 (135Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "Eravins",
+ "coords": {
+ "x": -32.84375,
+ "y": -253.96875,
+ "z": 27.15625
+ }
+ }
+ },
+ {
+ "title": "Jicasses - HR 32 (169Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Jicasses",
+ "coords": {
+ "x": 132.09375,
+ "y": -251.5625,
+ "z": -42.9375
+ }
+ }
+ },
+ {
+ "title": "Tsakapai - HR 32 (111Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Tsakapai",
+ "coords": {
+ "x": 148.4375,
+ "y": -263.90625,
+ "z": 66.875
+ }
+ }
+ },
+ {
+ "title": "HIP 116066 - Col 285 Sector YU-F c11-1 (99Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 116066",
+ "coords": {
+ "x": 147.59375,
+ "y": -174.8125,
+ "z": 124.15625
+ }
+ }
+ },
+ {
+ "title": "HIP 104349 - Col 285 Sector YU-F c11-1 (95Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "HIP 104349",
+ "coords": {
+ "x": 146.625,
+ "y": -148.9375,
+ "z": 157.21875
+ }
+ }
+ },
+ {
+ "title": "Pienapa - ICZ ZK-X b1-0 (163Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "Pienapa",
+ "coords": {
+ "x": 77.0625,
+ "y": -258.375,
+ "z": 17.21875
+ }
+ }
+ },
+ {
+ "title": "Motilekui - HR 32 (186Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Motilekui",
+ "coords": {
+ "x": 115.9375,
+ "y": -244.65625,
+ "z": -64.875
+ }
+ }
+ },
+ {
+ "title": "Vasuki - HR 32 (99Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Vasuki",
+ "coords": {
+ "x": 101.40625,
+ "y": -239.71875,
+ "z": 51.25
+ }
+ }
+ },
+ {
+ "title": "Yen Ku - ICZ ZK-X b1-0 (160Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "Yen Ku",
+ "coords": {
+ "x": 72.71875,
+ "y": -255.71875,
+ "z": 33.1875
+ }
+ }
+ },
+ {
+ "title": "Gaunetet - Col 285 Sector YU-F c11-1 (164Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Gaunetet",
+ "coords": {
+ "x": 81.6875,
+ "y": -255.75,
+ "z": 102.6875
+ }
+ }
+ },
+ {
+ "title": "Jandini - HR 32 (137Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Jandini",
+ "coords": {
+ "x": 136.4375,
+ "y": -251.625,
+ "z": -1.625
+ }
+ }
+ },
+ {
+ "title": "Uibuth - Col 285 Sector YU-F c11-1 (114Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Uibuth",
+ "coords": {
+ "x": 140.8125,
+ "y": -192.96875,
+ "z": 131.1875
+ }
+ }
+ },
+ {
+ "title": "Wurun - HR 32 (93Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Wurun",
+ "coords": {
+ "x": 221.78125,
+ "y": -157.6875,
+ "z": 73.84375
+ }
+ }
+ },
+ {
+ "title": "Vettorasare - HR 32 (154Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Vettorasare",
+ "coords": {
+ "x": 129.40625,
+ "y": -260.0,
+ "z": -17.03125
+ }
+ }
+ },
+ {
+ "title": "Khamataman - ICZ ZK-X b1-0 (144Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "Khamataman",
+ "coords": {
+ "x": 55.21875,
+ "y": -242.65625,
+ "z": -30.3125
+ }
+ }
+ },
+ {
+ "title": "Seliu Benas - Hyades Sector LC-L b8-0 (112Ly)",
+ "prison": {
+ "name": "Hyades Sector LC-L b8-0",
+ "coords": {
+ "x": 93.71875,
+ "y": -103.625,
+ "z": -85.9375
+ }
+ },
+ "jump": {
+ "name": "Seliu Benas",
+ "coords": {
+ "x": 130.8125,
+ "y": -208.8125,
+ "z": -95.9375
+ }
+ }
+ },
+ {
+ "title": "Karunti - HR 32 (83Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Karunti",
+ "coords": {
+ "x": 132.5625,
+ "y": -240.53125,
+ "z": 115.5
+ }
+ }
+ },
+ {
+ "title": "Rohini - Nyeajaae VU-Y a27-9 (7Ly)",
+ "prison": {
+ "name": "Nyeajaae VU-Y a27-9",
+ "coords": {
+ "x": -3374,
+ "y": -42.375,
+ "z": 6907.96875
+ }
+ },
+ "jump": {
+ "name": "Rohini",
+ "coords": {
+ "x": -3374.8125,
+ "y": -47.8125,
+ "z": 6912.25
+ }
+ }
+ },
+ {
+ "title": "Pleiades Sector HR-W d1-74 - Pleiades Sector HR-W d1-79 (8Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Pleiades Sector HR-W d1-74",
+ "coords": {
+ "x": -87.40625,
+ "y": -150.90625,
+ "z": -344.96875
+ }
+ }
+ },
+ {
+ "title": "Kurine - Hyades Sector LC-L b8-0 (160Ly)",
+ "prison": {
+ "name": "Hyades Sector LC-L b8-0",
+ "coords": {
+ "x": 93.71875,
+ "y": -103.625,
+ "z": -85.9375
+ }
+ },
+ "jump": {
+ "name": "Kurine",
+ "coords": {
+ "x": 77.9375,
+ "y": -252.40625,
+ "z": -28.46875
+ }
+ }
+ },
+ {
+ "title": "Maker - Synuefe BR-L c24-24 (113Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Maker",
+ "coords": {
+ "x": 157.875,
+ "y": -207.625,
+ "z": 33.84375
+ }
+ }
+ },
+ {
+ "title": "Aeducini - Synuefe BR-L c24-24 (59Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Aeducini",
+ "coords": {
+ "x": 197.78125,
+ "y": -148.3125,
+ "z": 37.8125
+ }
+ }
+ },
+ {
+ "title": "Bleia Dryiae HK-Y c17-9 - Omega Sector OD-S b4-0 (1016Ly)",
+ "prison": {
+ "name": "Omega Sector OD-S b4-0",
+ "coords": {
+ "x": -1456.8125,
+ "y": -84.8125,
+ "z": 5306.9375
+ }
+ },
+ "jump": {
+ "name": "Bleia Dryiae HK-Y c17-9",
+ "coords": {
+ "x": -553.875,
+ "y": -52.6875,
+ "z": 4843.21875
+ }
+ }
+ },
+ {
+ "title": "Shu Babassi - ICZ KS-T b3-5 (123Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Shu Babassi",
+ "coords": {
+ "x": -2.5625,
+ "y": -264.46875,
+ "z": 22.65625
+ }
+ }
+ },
+ {
+ "title": "Moyot - ICZ KS-T b3-5 (124Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Moyot",
+ "coords": {
+ "x": 24.8125,
+ "y": -260.65625,
+ "z": 12.78125
+ }
+ }
+ },
+ {
+ "title": "Gundji - ICZ ZK-X b1-0 (142Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "Gundji",
+ "coords": {
+ "x": 43.8125,
+ "y": -249.65625,
+ "z": 36.75
+ }
+ }
+ },
+ {
+ "title": "Potilla - Hyades Sector LC-L b8-0 (169Ly)",
+ "prison": {
+ "name": "Hyades Sector LC-L b8-0",
+ "coords": {
+ "x": 93.71875,
+ "y": -103.625,
+ "z": -85.9375
+ }
+ },
+ "jump": {
+ "name": "Potilla",
+ "coords": {
+ "x": 73.34375,
+ "y": -262.65625,
+ "z": -33.6875
+ }
+ }
+ },
+ {
+ "title": "Joku - Col 285 Sector YU-F c11-1 (151Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Joku",
+ "coords": {
+ "x": 76.875,
+ "y": -238.0,
+ "z": 64.71875
+ }
+ }
+ },
+ {
+ "title": "Mawula - HR 32 (107Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Mawula",
+ "coords": {
+ "x": 114.28125,
+ "y": -248.0,
+ "z": 41.5
+ }
+ }
+ },
+ {
+ "title": "Ladagao - HR 32 (90Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Ladagao",
+ "coords": {
+ "x": 128.0,
+ "y": -248.59375,
+ "z": 90.4375
+ }
+ }
+ },
+ {
+ "title": "Astrimui - Col 285 Sector YU-F c11-1 (119Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Astrimui",
+ "coords": {
+ "x": 122.03125,
+ "y": -203.4375,
+ "z": 134.53125
+ }
+ }
+ },
+ {
+ "title": "Tojo - Col 285 Sector YU-F c11-1 (97Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Tojo",
+ "coords": {
+ "x": 103.875,
+ "y": -181.125,
+ "z": 137.5625
+ }
+ }
+ },
+ {
+ "title": "Aracenth - Synuefe BR-L c24-24 (113Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Aracenth",
+ "coords": {
+ "x": 147.125,
+ "y": -207.9375,
+ "z": -2.53125
+ }
+ }
+ },
+ {
+ "title": "Luch - Synuefe BR-L c24-24 (46Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Luch",
+ "coords": {
+ "x": 204.5625,
+ "y": -168.875,
+ "z": -12.09375
+ }
+ }
+ },
+ {
+ "title": "Haki - Bei Dou Sector DL-Y d76 (935Ly)",
+ "prison": {
+ "name": "Bei Dou Sector DL-Y d76",
+ "coords": {
+ "x": -37.6875,
+ "y": 70.09375,
+ "z": -14.1875
+ }
+ },
+ "jump": {
+ "name": "Haki",
+ "coords": {
+ "x": 388.0625,
+ "y": -395.03125,
+ "z": -705.125
+ }
+ }
+ },
+ {
+ "title": "Ogones - ICZ KS-T b3-5 (139Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Ogones",
+ "coords": {
+ "x": 81.8125,
+ "y": -242.65625,
+ "z": -4.84375
+ }
+ }
+ },
+ {
+ "title": "Ngomangatio - HR 32 (104Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Ngomangatio",
+ "coords": {
+ "x": 58.21875,
+ "y": -232.0625,
+ "z": 104.25
+ }
+ }
+ },
+ {
+ "title": "Muthnir - Synuefe BR-L c24-24 (115Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Muthnir",
+ "coords": {
+ "x": 184.75,
+ "y": -176.6875,
+ "z": 87.375
+ }
+ }
+ },
+ {
+ "title": "Chaitany - ICZ ZK-X b1-0 (147Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "Chaitany",
+ "coords": {
+ "x": 44.53125,
+ "y": -235.09375,
+ "z": 86.75
+ }
+ }
+ },
+ {
+ "title": "Volupana - Synuefe BR-L c24-24 (101Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Volupana",
+ "coords": {
+ "x": 156.03125,
+ "y": -200.6875,
+ "z": -1.5
+ }
+ }
+ },
+ {
+ "title": "Acani - Synuefe BR-L c24-24 (61Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Acani",
+ "coords": {
+ "x": 189.90625,
+ "y": -173.96875,
+ "z": 9.96875
+ }
+ }
+ },
+ {
+ "title": "Cayu - ICZ KS-T b3-5 (124Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Cayu",
+ "coords": {
+ "x": 33.5625,
+ "y": -261.5,
+ "z": 35.25
+ }
+ }
+ },
+ {
+ "title": "Jagai - HR 32 (115Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Jagai",
+ "coords": {
+ "x": 59.90625,
+ "y": -240.8125,
+ "z": 63.625
+ }
+ }
+ },
+ {
+ "title": "Caret Werek - Synuefe BR-L c24-24 (56Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Caret Werek",
+ "coords": {
+ "x": 206.125,
+ "y": -178.5,
+ "z": -24.96875
+ }
+ }
+ },
+ {
+ "title": "Coelachi - HR 32 (49Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Coelachi",
+ "coords": {
+ "x": 178.25,
+ "y": -173.6875,
+ "z": 109.8125
+ }
+ }
+ },
+ {
+ "title": "Randgnid - Eol Prou LW-L c8-127 (30Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Randgnid",
+ "coords": {
+ "x": -9547.125,
+ "y": -916.5,
+ "z": 19832.5
+ }
+ }
+ },
+ {
+ "title": "Almagest - Pleiades Sector HR-W d1-79 (248Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Almagest",
+ "coords": {
+ "x": -274.65625,
+ "y": -10.8125,
+ "z": -271.34375
+ }
+ }
+ },
+ {
+ "title": "Rangda - HR 32 (153Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Rangda",
+ "coords": {
+ "x": 94.25,
+ "y": -241.0625,
+ "z": -24.03125
+ }
+ }
+ },
+ {
+ "title": "Fo Hi - ICZ KS-T b3-5 (124Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Fo Hi",
+ "coords": {
+ "x": 41.1875,
+ "y": -257.90625,
+ "z": 60.0
+ }
+ }
+ },
+ {
+ "title": "Ingui - Synuefe BR-L c24-24 (50Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Ingui",
+ "coords": {
+ "x": 215.40625,
+ "y": -171.09375,
+ "z": 17.28125
+ }
+ }
+ },
+ {
+ "title": "Alberta - Eol Prou LW-L c8-127 (19Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Alberta",
+ "coords": {
+ "x": -9536.96875,
+ "y": -917.25,
+ "z": 19793.03125
+ }
+ }
+ },
+ {
+ "title": "Tenjin - Eol Prou LW-L c8-127 (11Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Tenjin",
+ "coords": {
+ "x": -9539.4375,
+ "y": -910.0,
+ "z": 19812.28125
+ }
+ }
+ },
+ {
+ "title": "Far Tauri - Eol Prou LW-L c8-127 (24Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Far Tauri",
+ "coords": {
+ "x": -9510.65625,
+ "y": -915.0,
+ "z": 19793.34375
+ }
+ }
+ },
+ {
+ "title": "Shorodo - HR 32 (98Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Shorodo",
+ "coords": {
+ "x": 159.40625,
+ "y": -253.5625,
+ "z": 99.3125
+ }
+ }
+ },
+ {
+ "title": "Apotanites - Col 285 Sector YU-F c11-1 (113Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Apotanites",
+ "coords": {
+ "x": 102.25,
+ "y": -159.75,
+ "z": 188.21875
+ }
+ }
+ },
+ {
+ "title": "Zende - Synuefe BR-L c24-24 (9Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Zende",
+ "coords": {
+ "x": 226.0625,
+ "y": -134.3125,
+ "z": -1.75
+ }
+ }
+ },
+ {
+ "title": "Dubbuennel - Eol Prou LW-L c8-127 (24Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Dubbuennel",
+ "coords": {
+ "x": -9521.40625,
+ "y": -913.5625,
+ "z": 19786.53125
+ }
+ }
+ },
+ {
+ "title": "Arikudiil - Col 285 Sector YU-F c11-1 (132Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Arikudiil",
+ "coords": {
+ "x": 111.8125,
+ "y": -222.9375,
+ "z": 85.84375
+ }
+ }
+ },
+ {
+ "title": "Yawalapiti - Synuefe BR-L c24-24 (92Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Yawalapiti",
+ "coords": {
+ "x": 203.75,
+ "y": -173.75,
+ "z": 68.125
+ }
+ }
+ },
+ {
+ "title": "Blu Thua AI-A c14-10 - Alrai Sector KN-S b4-4 (1999Ly)",
+ "prison": {
+ "name": "Alrai Sector KN-S b4-4",
+ "coords": {
+ "x": -34.96875,
+ "y": 77.625,
+ "z": 101.59375
+ }
+ },
+ "jump": {
+ "name": "Blu Thua AI-A c14-10",
+ "coords": {
+ "x": -54.5,
+ "y": 149.53125,
+ "z": 2099.21875
+ }
+ }
+ },
+ {
+ "title": "Tacabs - ICZ KS-T b3-5 (125Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Tacabs",
+ "coords": {
+ "x": 20.21875,
+ "y": -261.90625,
+ "z": 9.96875
+ }
+ }
+ },
+ {
+ "title": "Nahuahini - HR 32 (121Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Nahuahini",
+ "coords": {
+ "x": 53.84375,
+ "y": -248.84375,
+ "z": 78.65625
+ }
+ }
+ },
+ {
+ "title": "Ajoku - Hyades Sector LC-L b8-0 (153Ly)",
+ "prison": {
+ "name": "Hyades Sector LC-L b8-0",
+ "coords": {
+ "x": 93.71875,
+ "y": -103.625,
+ "z": -85.9375
+ }
+ },
+ "jump": {
+ "name": "Ajoku",
+ "coords": {
+ "x": 56.46875,
+ "y": -250.0625,
+ "z": -63.84375
+ }
+ }
+ },
+ {
+ "title": "Sagartiaci - ICZ KS-T b3-5 (142Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Sagartiaci",
+ "coords": {
+ "x": 56.5625,
+ "y": -255.03125,
+ "z": -21.15625
+ }
+ }
+ },
+ {
+ "title": "Garma - Col 285 Sector YU-F c11-1 (155Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Garma",
+ "coords": {
+ "x": 78.09375,
+ "y": -241.8125,
+ "z": 62.375
+ }
+ }
+ },
+ {
+ "title": "Zaparo - HR 32 (145Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Zaparo",
+ "coords": {
+ "x": 122.84375,
+ "y": -246.40625,
+ "z": -16.8125
+ }
+ }
+ },
+ {
+ "title": "Humana - HR 32 (106Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Humana",
+ "coords": {
+ "x": 116.75,
+ "y": -245.4375,
+ "z": 40.3125
+ }
+ }
+ },
+ {
+ "title": "Cant - HR 32 (91Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Cant",
+ "coords": {
+ "x": 126.40625,
+ "y": -249.03125,
+ "z": 87.78125
+ }
+ }
+ },
+ {
+ "title": "Serd - Synuefe BR-L c24-24 (109Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Serd",
+ "coords": {
+ "x": 151.0,
+ "y": -194.0,
+ "z": -50.125
+ }
+ }
+ },
+ {
+ "title": "Mompox - HR 32 (114Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Mompox",
+ "coords": {
+ "x": 138.9375,
+ "y": -213.9375,
+ "z": -0.8125
+ }
+ }
+ },
+ {
+ "title": "Tehuencho - Synuefe BR-L c24-24 (122Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Tehuencho",
+ "coords": {
+ "x": 164.28125,
+ "y": -221.65625,
+ "z": 39.0625
+ }
+ }
+ },
+ {
+ "title": "Bafook - Synuefe BR-L c24-24 (126Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Bafook",
+ "coords": {
+ "x": 165.3125,
+ "y": -199.84375,
+ "z": 75.25
+ }
+ }
+ },
+ {
+ "title": "Khemaraui - Synuefe BR-L c24-24 (108Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Khemaraui",
+ "coords": {
+ "x": 203.0625,
+ "y": -175.28125,
+ "z": 85.5625
+ }
+ }
+ },
+ {
+ "title": "Siniang - Bei Dou Sector DL-Y d76 (919Ly)",
+ "prison": {
+ "name": "Bei Dou Sector DL-Y d76",
+ "coords": {
+ "x": -37.6875,
+ "y": 70.09375,
+ "z": -14.1875
+ }
+ },
+ "jump": {
+ "name": "Siniang",
+ "coords": {
+ "x": 354.40625,
+ "y": -386.625,
+ "z": -709.21875
+ }
+ }
+ },
+ {
+ "title": "Wellington - Bei Dou Sector DL-Y d76 (923Ly)",
+ "prison": {
+ "name": "Bei Dou Sector DL-Y d76",
+ "coords": {
+ "x": -37.6875,
+ "y": 70.09375,
+ "z": -14.1875
+ }
+ },
+ "jump": {
+ "name": "Wellington",
+ "coords": {
+ "x": 386.15625,
+ "y": -390.75,
+ "z": -692.53125
+ }
+ }
+ },
+ {
+ "title": "Onuro - HR 32 (60Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Onuro",
+ "coords": {
+ "x": 111.4375,
+ "y": -214.46875,
+ "z": 88.5625
+ }
+ }
+ },
+ {
+ "title": "Belinichs - HR 32 (76Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Belinichs",
+ "coords": {
+ "x": 194.59375,
+ "y": -171.75,
+ "z": 56.34375
+ }
+ }
+ },
+ {
+ "title": "IC 1287 Sector RO-Q b5-1 - Capricorni Sector FM-V b2-0 (886Ly)",
+ "prison": {
+ "name": "Capricorni Sector FM-V b2-0",
+ "coords": {
+ "x": -103.59375,
+ "y": -100.375,
+ "z": 97.4375
+ }
+ },
+ "jump": {
+ "name": "IC 1287 Sector RO-Q b5-1",
+ "coords": {
+ "x": -364.5,
+ "y": -3.1875,
+ "z": 938.96875
+ }
+ }
+ },
+ {
+ "title": "Smojue IY-Q b32-1 - Omega Sector OD-S b4-0 (2215Ly)",
+ "prison": {
+ "name": "Omega Sector OD-S b4-0",
+ "coords": {
+ "x": -1456.8125,
+ "y": -84.8125,
+ "z": 5306.9375
+ }
+ },
+ "jump": {
+ "name": "Smojue IY-Q b32-1",
+ "coords": {
+ "x": -207.78125,
+ "y": 39.03125,
+ "z": 3481.59375
+ }
+ }
+ },
+ {
+ "title": "Bleae Thua WD-M b49-1 - Alrai Sector KN-S b4-4 (2457Ly)",
+ "prison": {
+ "name": "Alrai Sector KN-S b4-4",
+ "coords": {
+ "x": -34.96875,
+ "y": 77.625,
+ "z": 101.59375
+ }
+ },
+ "jump": {
+ "name": "Bleae Thua WD-M b49-1",
+ "coords": {
+ "x": -127.78125,
+ "y": 146.03125,
+ "z": 2556.125
+ }
+ }
+ },
+ {
+ "title": "Maria Bhuti - ICZ KS-T b3-5 (116Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Maria Bhuti",
+ "coords": {
+ "x": 60.03125,
+ "y": -240.96875,
+ "z": 41.15625
+ }
+ }
+ },
+ {
+ "title": "Skil'slandi - ICZ ZK-X b1-0 (145Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "Skil'slandi",
+ "coords": {
+ "x": 62.125,
+ "y": -232.28125,
+ "z": 69.5625
+ }
+ }
+ },
+ {
+ "title": "Scirtari - Col 285 Sector YU-F c11-1 (143Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Scirtari",
+ "coords": {
+ "x": 68.6875,
+ "y": -231.625,
+ "z": 78.3125
+ }
+ }
+ },
+ {
+ "title": "Kawal - Col 285 Sector YU-F c11-1 (140Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Kawal",
+ "coords": {
+ "x": 56.5625,
+ "y": -222.65625,
+ "z": 127.28125
+ }
+ }
+ },
+ {
+ "title": "Warii - ICZ ZK-X b1-0 (150Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "Warii",
+ "coords": {
+ "x": 86.0,
+ "y": -234.59375,
+ "z": 29.34375
+ }
+ }
+ },
+ {
+ "title": "Lobetati - Col 285 Sector YU-F c11-1 (156Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Lobetati",
+ "coords": {
+ "x": 83.375,
+ "y": -242.71875,
+ "z": 58.75
+ }
+ }
+ },
+ {
+ "title": "Chak - Col 285 Sector YU-F c11-1 (126Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Chak",
+ "coords": {
+ "x": 82.1875,
+ "y": -216.90625,
+ "z": 113.25
+ }
+ }
+ },
+ {
+ "title": "Biambar - Synuefe BR-L c24-24 (165Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Biambar",
+ "coords": {
+ "x": 112.5,
+ "y": -241.90625,
+ "z": 25.5625
+ }
+ }
+ },
+ {
+ "title": "Cantei - Col 285 Sector YU-F c11-1 (125Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Cantei",
+ "coords": {
+ "x": 107.59375,
+ "y": -217.09375,
+ "z": 95.75
+ }
+ }
+ },
+ {
+ "title": "Selkit - HR 32 (131Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Selkit",
+ "coords": {
+ "x": 118.9375,
+ "y": -240.9375,
+ "z": -2.46875
+ }
+ }
+ },
+ {
+ "title": "Padh - HR 32 (91Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Padh",
+ "coords": {
+ "x": 119.75,
+ "y": -241.96875,
+ "z": 63.34375
+ }
+ }
+ },
+ {
+ "title": "Wikmung - HR 32 (86Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Wikmung",
+ "coords": {
+ "x": 116.6875,
+ "y": -240.75,
+ "z": 76.3125
+ }
+ }
+ },
+ {
+ "title": "Fan Chaguti - Hyades Sector LC-L b8-0 (128Ly)",
+ "prison": {
+ "name": "Hyades Sector LC-L b8-0",
+ "coords": {
+ "x": 93.71875,
+ "y": -103.625,
+ "z": -85.9375
+ }
+ },
+ "jump": {
+ "name": "Fan Chaguti",
+ "coords": {
+ "x": 115.125,
+ "y": -222.15625,
+ "z": -42.625
+ }
+ }
+ },
+ {
+ "title": "Jagaluk - HR 32 (136Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Jagaluk",
+ "coords": {
+ "x": 130.3125,
+ "y": -215.84375,
+ "z": -24.125
+ }
+ }
+ },
+ {
+ "title": "Gandoques - Col 285 Sector YU-F c11-1 (125Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Gandoques",
+ "coords": {
+ "x": 118.59375,
+ "y": -215.90625,
+ "z": 86.84375
+ }
+ }
+ },
+ {
+ "title": "Juato - Col 285 Sector YU-F c11-1 (95Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Juato",
+ "coords": {
+ "x": 123.28125,
+ "y": -177.34375,
+ "z": 132.3125
+ }
+ }
+ },
+ {
+ "title": "Cile - Col 285 Sector YU-F c11-1 (98Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Cile",
+ "coords": {
+ "x": 135.3125,
+ "y": -179.21875,
+ "z": 123.25
+ }
+ }
+ },
+ {
+ "title": "Skoko - Synuefe BR-L c24-24 (126Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Skoko",
+ "coords": {
+ "x": 168.03125,
+ "y": -222.375,
+ "z": 51.53125
+ }
+ }
+ },
+ {
+ "title": "Koartoq - HR 32 (128Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Koartoq",
+ "coords": {
+ "x": 174.65625,
+ "y": -203.96875,
+ "z": -12.90625
+ }
+ }
+ },
+ {
+ "title": "Madngenses - Synuefe BR-L c24-24 (107Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Madngenses",
+ "coords": {
+ "x": 155.46875,
+ "y": -204.40625,
+ "z": 16.34375
+ }
+ }
+ },
+ {
+ "title": "Auso - HR 32 (50Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Auso",
+ "coords": {
+ "x": 164.25,
+ "y": -194.90625,
+ "z": 85.53125
+ }
+ }
+ },
+ {
+ "title": "Selkadiae - HR 32 (47Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Selkadiae",
+ "coords": {
+ "x": 172.09375,
+ "y": -180.625,
+ "z": 86.15625
+ }
+ }
+ },
+ {
+ "title": "Nitikudi - HR 32 (75Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Nitikudi",
+ "coords": {
+ "x": 175.46875,
+ "y": -179.34375,
+ "z": 41.6875
+ }
+ }
+ },
+ {
+ "title": "Musca Dark Region PJ-P b6-1 - Crucis Sector ND-S b4-5 (414Ly)",
+ "prison": {
+ "name": "Crucis Sector ND-S b4-5",
+ "coords": {
+ "x": 78.6875,
+ "y": 53.9375,
+ "z": 80.03125
+ }
+ },
+ "jump": {
+ "name": "Musca Dark Region PJ-P b6-1",
+ "coords": {
+ "x": 432.625,
+ "y": 2.53125,
+ "z": 288.6875
+ }
+ }
+ },
+ {
+ "title": "Vesuvit - HR 32 (81Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Vesuvit",
+ "coords": {
+ "x": 145.09375,
+ "y": -239.5625,
+ "z": 97.4375
+ }
+ }
+ },
+ {
+ "title": "Ithel - HR 32 (72Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Ithel",
+ "coords": {
+ "x": 188.40625,
+ "y": -180.46875,
+ "z": 59.84375
+ }
+ }
+ },
+ {
+ "title": "Nyament - Col 285 Sector YU-F c11-1 (157Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Nyament",
+ "coords": {
+ "x": 101.90625,
+ "y": -246.8125,
+ "z": 129.34375
+ }
+ }
+ },
+ {
+ "title": "Hsuan Zu - Col 285 Sector YU-F c11-1 (155Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Hsuan Zu",
+ "coords": {
+ "x": 114.3125,
+ "y": -246.28125,
+ "z": 110.09375
+ }
+ }
+ },
+ {
+ "title": "Hillaunges - HR 32 (80Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Hillaunges",
+ "coords": {
+ "x": 206.375,
+ "y": -131.90625,
+ "z": 83.21875
+ }
+ }
+ },
+ {
+ "title": "Pleiades Sector KC-V c2-11 - Pleiades Sector HR-W d1-79 (7Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Pleiades Sector KC-V c2-11",
+ "coords": {
+ "x": -86.59375,
+ "y": -147.03125,
+ "z": -339.28125
+ }
+ }
+ },
+ {
+ "title": "Kats - ICZ KS-T b3-5 (108Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Kats",
+ "coords": {
+ "x": 25.875,
+ "y": -238.9375,
+ "z": 84.03125
+ }
+ }
+ },
+ {
+ "title": "Dijuhte - ICZ KS-T b3-5 (145Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Dijuhte",
+ "coords": {
+ "x": 65.0625,
+ "y": -251.21875,
+ "z": -25.59375
+ }
+ }
+ },
+ {
+ "title": "Lwendecht - Col 285 Sector YU-F c11-1 (169Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Lwendecht",
+ "coords": {
+ "x": 94.0625,
+ "y": -255.21875,
+ "z": 53.375
+ }
+ }
+ },
+ {
+ "title": "Angokomu - Col 285 Sector YU-F c11-1 (157Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Angokomu",
+ "coords": {
+ "x": 76.25,
+ "y": -244.5,
+ "z": 65.25
+ }
+ }
+ },
+ {
+ "title": "Bende - HR 32 (85Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Bende",
+ "coords": {
+ "x": 131.0625,
+ "y": -240.875,
+ "z": 74.6875
+ }
+ }
+ },
+ {
+ "title": "Arapani - Col 285 Sector YU-F c11-1 (120Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Arapani",
+ "coords": {
+ "x": 119.84375,
+ "y": -208.1875,
+ "z": 123.8125
+ }
+ }
+ },
+ {
+ "title": "Czerni - HR 32 (110Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Czerni",
+ "coords": {
+ "x": 137.09375,
+ "y": -209.21875,
+ "z": 1.09375
+ }
+ }
+ },
+ {
+ "title": "Halanduni - HR 32 (91Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Halanduni",
+ "coords": {
+ "x": 164.5625,
+ "y": -218.28125,
+ "z": 37.0625
+ }
+ }
+ },
+ {
+ "title": "Chet - Col 285 Sector YU-F c11-1 (114Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Chet",
+ "coords": {
+ "x": 138.6875,
+ "y": -195.65625,
+ "z": 126.46875
+ }
+ }
+ },
+ {
+ "title": "Taurt - HR 32 (93Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Taurt",
+ "coords": {
+ "x": 211.34375,
+ "y": -170.125,
+ "z": 51.84375
+ }
+ }
+ },
+ {
+ "title": "Ngongani - Col 285 Sector YU-F c11-1 (104Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Ngongani",
+ "coords": {
+ "x": 195.625,
+ "y": -129.6875,
+ "z": 94.3125
+ }
+ }
+ },
+ {
+ "title": "Onoros - HR 32 (890Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Onoros",
+ "coords": {
+ "x": 389.71875,
+ "y": -379.9375,
+ "z": -724.15625
+ }
+ }
+ },
+ {
+ "title": "Sothis - G 203-51 (487Ly)",
+ "prison": {
+ "name": "G 203-51",
+ "coords": {
+ "x": -15.875,
+ "y": 12.03125,
+ "z": 5.34375
+ }
+ },
+ "jump": {
+ "name": "Sothis",
+ "coords": {
+ "x": -352.78125,
+ "y": 10.5,
+ "z": -346.34375
+ }
+ }
+ },
+ {
+ "title": "Mexicatuci - Col 285 Sector YU-F c11-1 (88Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Mexicatuci",
+ "coords": {
+ "x": 158.90625,
+ "y": -145.65625,
+ "z": 134.1875
+ }
+ }
+ },
+ {
+ "title": "Ualarni - Synuefe BR-L c24-24 (56Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Ualarni",
+ "coords": {
+ "x": 214.5625,
+ "y": -174.34375,
+ "z": 22.25
+ }
+ }
+ },
+ {
+ "title": "Muthia - Synuefe BR-L c24-24 (92Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Muthia",
+ "coords": {
+ "x": 205.59375,
+ "y": -170.875,
+ "z": 70.90625
+ }
+ }
+ },
+ {
+ "title": "Colonia - Eol Prou LW-L c8-127 (4Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Colonia",
+ "coords": {
+ "x": -9530.5,
+ "y": -910.28125,
+ "z": 19808.125
+ }
+ }
+ },
+ {
+ "title": "Khakya - Col 285 Sector YU-F c11-1 (106Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Khakya",
+ "coords": {
+ "x": 192.875,
+ "y": -140.15625,
+ "z": 96.53125
+ }
+ }
+ },
+ {
+ "title": "Synuefe EN-H d11-96 - HR 3005 (13Ly)",
+ "prison": {
+ "name": "HR 3005",
+ "coords": {
+ "x": 750.53125,
+ "y": -169.65625,
+ "z": -90
+ }
+ },
+ "jump": {
+ "name": "Synuefe EN-H d11-96",
+ "coords": {
+ "x": 757.125,
+ "y": -179.3125,
+ "z": -96.0625
+ }
+ }
+ },
+ {
+ "title": "Synuefe BT-F d12-97 - Synuefe BR-L c24-24 (41Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Synuefe BT-F d12-97",
+ "coords": {
+ "x": 221.375,
+ "y": -115.1875,
+ "z": 28.625
+ }
+ }
+ },
+ {
+ "title": "Kokoja - HR 32 (76Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Kokoja",
+ "coords": {
+ "x": 182.375,
+ "y": -169.0625,
+ "z": 43.21875
+ }
+ }
+ },
+ {
+ "title": "Bleae Thua KY-L c7-12 - Alrai Sector KN-S b4-4 (1748Ly)",
+ "prison": {
+ "name": "Alrai Sector KN-S b4-4",
+ "coords": {
+ "x": -34.96875,
+ "y": 77.625,
+ "z": 101.59375
+ }
+ },
+ "jump": {
+ "name": "Bleae Thua KY-L c7-12",
+ "coords": {
+ "x": -132.0625,
+ "y": -7.4375,
+ "z": 1844.75
+ }
+ }
+ },
+ {
+ "title": "Awabakal - ICZ KS-T b3-5 (103Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Awabakal",
+ "coords": {
+ "x": 19.28125,
+ "y": -236.21875,
+ "z": 81.21875
+ }
+ }
+ },
+ {
+ "title": "Bunbudo - ICZ KS-T b3-5 (145Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Bunbudo",
+ "coords": {
+ "x": 65.71875,
+ "y": -260.84375,
+ "z": -7.4375
+ }
+ }
+ },
+ {
+ "title": "Dhakua - Col 285 Sector YU-F c11-1 (113Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Dhakua",
+ "coords": {
+ "x": 89.78125,
+ "y": -182.375,
+ "z": 165.84375
+ }
+ }
+ },
+ {
+ "title": "Palliep - HR 32 (152Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Palliep",
+ "coords": {
+ "x": 117.46875,
+ "y": -252.90625,
+ "z": -19.6875
+ }
+ }
+ },
+ {
+ "title": "Iconti Shi - HR 32 (109Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Iconti Shi",
+ "coords": {
+ "x": 115.71875,
+ "y": -243.96875,
+ "z": 32.96875
+ }
+ }
+ },
+ {
+ "title": "Tripurus - Col 285 Sector YU-F c11-1 (97Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Tripurus",
+ "coords": {
+ "x": 104.40625,
+ "y": -179.1875,
+ "z": 140.9375
+ }
+ }
+ },
+ {
+ "title": "Njemar - Synuefe BR-L c24-24 (115Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Njemar",
+ "coords": {
+ "x": 157.34375,
+ "y": -204.90625,
+ "z": -60.375
+ }
+ }
+ },
+ {
+ "title": "Wulgu - Synuefe BR-L c24-24 (124Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Wulgu",
+ "coords": {
+ "x": 150.9375,
+ "y": -207.15625,
+ "z": 48.84375
+ }
+ }
+ },
+ {
+ "title": "Caeriango - Col 285 Sector YU-F c11-1 (98Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Caeriango",
+ "coords": {
+ "x": 138.375,
+ "y": -182.125,
+ "z": 102.78125
+ }
+ }
+ },
+ {
+ "title": "Yen Tacanik - Synuefe BR-L c24-24 (76Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Yen Tacanik",
+ "coords": {
+ "x": 207.625,
+ "y": -174.84375,
+ "z": 49.625
+ }
+ }
+ },
+ {
+ "title": "Walgalu - HR 32 (49Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Walgalu",
+ "coords": {
+ "x": 178.78125,
+ "y": -159.625,
+ "z": 83.59375
+ }
+ }
+ },
+ {
+ "title": "Otohime - HR 32 (64Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Otohime",
+ "coords": {
+ "x": 191.46875,
+ "y": -180.625,
+ "z": 88.96875
+ }
+ }
+ },
+ {
+ "title": "Bwenapok - HR 32 (101Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Bwenapok",
+ "coords": {
+ "x": 72.15625,
+ "y": -159.90625,
+ "z": 179.8125
+ }
+ }
+ },
+ {
+ "title": "Belinjaba - Col 285 Sector YU-F c11-1 (100Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Belinjaba",
+ "coords": {
+ "x": 160.25,
+ "y": -153.9375,
+ "z": 147.125
+ }
+ }
+ },
+ {
+ "title": "Eagle Sector IR-W d1-105 - Nyeajaae VU-Y a27-9 (1352Ly)",
+ "prison": {
+ "name": "Nyeajaae VU-Y a27-9",
+ "coords": {
+ "x": -3374,
+ "y": -42.375,
+ "z": 6907.96875
+ }
+ },
+ "jump": {
+ "name": "Eagle Sector IR-W d1-105",
+ "coords": {
+ "x": -2046.21875,
+ "y": 104.40625,
+ "z": 6699.90625
+ }
+ }
+ },
+ {
+ "title": "Pereng Asli - HR 32 (168Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Pereng Asli",
+ "coords": {
+ "x": 172.8125,
+ "y": -172.34375,
+ "z": -63.65625
+ }
+ }
+ },
+ {
+ "title": "Atese - HR 32 (120Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Atese",
+ "coords": {
+ "x": 76.28125,
+ "y": -179.5625,
+ "z": 203.4375
+ }
+ }
+ },
+ {
+ "title": "Pleiades Sector GW-W c1-13 - Pleiades Sector HR-W d1-79 (13Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Pleiades Sector GW-W c1-13",
+ "coords": {
+ "x": -77.375,
+ "y": -156.3125,
+ "z": -352.03125
+ }
+ }
+ },
+ {
+ "title": "Elycoco - ICZ ZK-X b1-0 (140Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "Elycoco",
+ "coords": {
+ "x": -18.84375,
+ "y": -261.65625,
+ "z": 15.71875
+ }
+ }
+ },
+ {
+ "title": "Shaplinouk - ICZ ZK-X b1-0 (137Ly)",
+ "prison": {
+ "name": "ICZ ZK-X b1-0",
+ "coords": {
+ "x": -10.96875,
+ "y": -121.5625,
+ "z": 11.4375
+ }
+ },
+ "jump": {
+ "name": "Shaplinouk",
+ "coords": {
+ "x": 47.375,
+ "y": -244.6875,
+ "z": 22.0
+ }
+ }
+ },
+ {
+ "title": "Tjupalar - ICZ KS-T b3-5 (104Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Tjupalar",
+ "coords": {
+ "x": 23.75,
+ "y": -236.84375,
+ "z": 80.40625
+ }
+ }
+ },
+ {
+ "title": "Gallemi - ICZ KS-T b3-5 (147Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Gallemi",
+ "coords": {
+ "x": 68.1875,
+ "y": -262.125,
+ "z": -5.5625
+ }
+ }
+ },
+ {
+ "title": "Gaarnakuruk - HR 32 (117Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Gaarnakuruk",
+ "coords": {
+ "x": 89.40625,
+ "y": -256.0,
+ "z": 50.40625
+ }
+ }
+ },
+ {
+ "title": "Eshu - HR 32 (146Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Eshu",
+ "coords": {
+ "x": 120.78125,
+ "y": -247.1875,
+ "z": -16.4375
+ }
+ }
+ },
+ {
+ "title": "Potrima - HR 32 (115Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Potrima",
+ "coords": {
+ "x": 118.46875,
+ "y": -250.28125,
+ "z": 31.09375
+ }
+ }
+ },
+ {
+ "title": "Bunbulama - HR 32 (76Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Bunbulama",
+ "coords": {
+ "x": 123.03125,
+ "y": -226.65625,
+ "z": 64.65625
+ }
+ }
+ },
+ {
+ "title": "Quillaente - Col 285 Sector YU-F c11-1 (100Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Quillaente",
+ "coords": {
+ "x": 98.375,
+ "y": -184.21875,
+ "z": 138.15625
+ }
+ }
+ },
+ {
+ "title": "Belung - HR 32 (112Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Belung",
+ "coords": {
+ "x": 140.21875,
+ "y": -209.5625,
+ "z": -0.46875
+ }
+ }
+ },
+ {
+ "title": "Hlocasses - Synuefe BR-L c24-24 (124Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Hlocasses",
+ "coords": {
+ "x": 149.8125,
+ "y": -209.21875,
+ "z": 46.1875
+ }
+ }
+ },
+ {
+ "title": "Auserid - HR 32 (60Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Auserid",
+ "coords": {
+ "x": 147.78125,
+ "y": -215.8125,
+ "z": 85.15625
+ }
+ }
+ },
+ {
+ "title": "Kurumian - Synuefe BR-L c24-24 (63Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Kurumian",
+ "coords": {
+ "x": 192.15625,
+ "y": -180.9375,
+ "z": -15.96875
+ }
+ }
+ },
+ {
+ "title": "Gurojas - Col 285 Sector YU-F c11-1 (108Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Gurojas",
+ "coords": {
+ "x": 179.0,
+ "y": -164.21875,
+ "z": 87.8125
+ }
+ }
+ },
+ {
+ "title": "Badar - Col 285 Sector YU-F c11-1 (102Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Badar",
+ "coords": {
+ "x": 165.65625,
+ "y": -165.5625,
+ "z": 121.6875
+ }
+ }
+ },
+ {
+ "title": "Bodhinga - Col 285 Sector YU-F c11-1 (104Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Bodhinga",
+ "coords": {
+ "x": 92.75,
+ "y": -134.03125,
+ "z": 193.125
+ }
+ }
+ },
+ {
+ "title": "Coniaci - Col 285 Sector YU-F c11-1 (100Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Coniaci",
+ "coords": {
+ "x": 174.71875,
+ "y": -155.625,
+ "z": 114.34375
+ }
+ }
+ },
+ {
+ "title": "Zagombo - Col 285 Sector YU-F c11-1 (104Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Zagombo",
+ "coords": {
+ "x": 139.53125,
+ "y": -178.0625,
+ "z": 141.125
+ }
+ }
+ },
+ {
+ "title": "Brigama - Col 285 Sector YU-F c11-1 (103Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Brigama",
+ "coords": {
+ "x": 90.75,
+ "y": -168.59375,
+ "z": 166.28125
+ }
+ }
+ },
+ {
+ "title": "Shillici - Synuefe BR-L c24-24 (61Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Shillici",
+ "coords": {
+ "x": 190.125,
+ "y": -177.4375,
+ "z": -2.28125
+ }
+ }
+ },
+ {
+ "title": "Naporimui - Col 285 Sector YU-F c11-1 (144Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Naporimui",
+ "coords": {
+ "x": 29.15625,
+ "y": -215.5625,
+ "z": 125.3125
+ }
+ }
+ },
+ {
+ "title": "Konejang - HR 32 (118Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Konejang",
+ "coords": {
+ "x": 87.71875,
+ "y": -257.875,
+ "z": 51.4375
+ }
+ }
+ },
+ {
+ "title": "Djabara - Col 285 Sector YU-F c11-1 (151Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Djabara",
+ "coords": {
+ "x": 90.28125,
+ "y": -237.4375,
+ "z": 57.25
+ }
+ }
+ },
+ {
+ "title": "Basterni - HR 32 (111Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Basterni",
+ "coords": {
+ "x": 111.25,
+ "y": -257.84375,
+ "z": 52.75
+ }
+ }
+ },
+ {
+ "title": "Sigue - Hyades Sector LC-L b8-0 (127Ly)",
+ "prison": {
+ "name": "Hyades Sector LC-L b8-0",
+ "coords": {
+ "x": 93.71875,
+ "y": -103.625,
+ "z": -85.9375
+ }
+ },
+ "jump": {
+ "name": "Sigue",
+ "coords": {
+ "x": 128.40625,
+ "y": -222.15625,
+ "z": -58.40625
+ }
+ }
+ },
+ {
+ "title": "Krinu - Col 285 Sector YU-F c11-1 (124Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Krinu",
+ "coords": {
+ "x": 106.4375,
+ "y": -216.46875,
+ "z": 103.96875
+ }
+ }
+ },
+ {
+ "title": "Akbakshu - Col 285 Sector YU-F c11-1 (112Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Akbakshu",
+ "coords": {
+ "x": 141.96875,
+ "y": -193.625,
+ "z": 120.03125
+ }
+ }
+ },
+ {
+ "title": "Nantoac - HR 32 (89Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Nantoac",
+ "coords": {
+ "x": 201.5,
+ "y": -177.8125,
+ "z": 46.9375
+ }
+ }
+ },
+ {
+ "title": "Boman - Synuefe BR-L c24-24 (105Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Boman",
+ "coords": {
+ "x": 200.53125,
+ "y": -153.3125,
+ "z": 89.78125
+ }
+ }
+ },
+ {
+ "title": "Xeret - Col 285 Sector YU-F c11-1 (113Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Xeret",
+ "coords": {
+ "x": 72.6875,
+ "y": -180.25,
+ "z": 164.5625
+ }
+ }
+ },
+ {
+ "title": "Bratadi - Synuefe BR-L c24-24 (103Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Bratadi",
+ "coords": {
+ "x": 202.90625,
+ "y": -146.75,
+ "z": 89.78125
+ }
+ }
+ },
+ {
+ "title": "Eagle Sector IR-W d1-117 - Nyeajaae VU-Y a27-9 (1341Ly)",
+ "prison": {
+ "name": "Nyeajaae VU-Y a27-9",
+ "coords": {
+ "x": -3374,
+ "y": -42.375,
+ "z": 6907.96875
+ }
+ },
+ "jump": {
+ "name": "Eagle Sector IR-W d1-117",
+ "coords": {
+ "x": -2054.09375,
+ "y": 85.71875,
+ "z": 6710.875
+ }
+ }
+ },
+ {
+ "title": "Singa - Synuefe BR-L c24-24 (103Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Singa",
+ "coords": {
+ "x": 204.59375,
+ "y": -155.5,
+ "z": 87.71875
+ }
+ }
+ },
+ {
+ "title": "Negri - Synuefe BR-L c24-24 (105Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Negri",
+ "coords": {
+ "x": 188.5,
+ "y": -173.0,
+ "z": 78.875
+ }
+ }
+ },
+ {
+ "title": "Shamaphan - Synuefe BR-L c24-24 (101Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Shamaphan",
+ "coords": {
+ "x": 179.21875,
+ "y": -164.90625,
+ "z": 71.875
+ }
+ }
+ },
+ {
+ "title": "Farwell - Eol Prou LW-L c8-127 (45Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Farwell",
+ "coords": {
+ "x": -9521.40625,
+ "y": -888.28125,
+ "z": 19845.65625
+ }
+ }
+ },
+ {
+ "title": "Morpheus - Eol Prou LW-L c8-127 (25Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Morpheus",
+ "coords": {
+ "x": -9543.96875,
+ "y": -892.9375,
+ "z": 19808.34375
+ }
+ }
+ },
+ {
+ "title": "Lagoon Sector FW-W d1-122 - Omega Sector OD-S b4-0 (1286Ly)",
+ "prison": {
+ "name": "Omega Sector OD-S b4-0",
+ "coords": {
+ "x": -1456.8125,
+ "y": -84.8125,
+ "z": 5306.9375
+ }
+ },
+ "jump": {
+ "name": "Lagoon Sector FW-W d1-122",
+ "coords": {
+ "x": -467.75,
+ "y": -93.1875,
+ "z": 4485.625
+ }
+ }
+ },
+ {
+ "title": "Iburri - Col 285 Sector YU-F c11-1 (96Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Iburri",
+ "coords": {
+ "x": 129.625,
+ "y": -166.5,
+ "z": 150.6875
+ }
+ }
+ },
+ {
+ "title": "Madyaksha - Col 285 Sector YU-F c11-1 (154Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Madyaksha",
+ "coords": {
+ "x": 89.625,
+ "y": -240.84375,
+ "z": 55.75
+ }
+ }
+ },
+ {
+ "title": "Hajanisei - HR 32 (111Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Hajanisei",
+ "coords": {
+ "x": 108.71875,
+ "y": -251.9375,
+ "z": 43.5625
+ }
+ }
+ },
+ {
+ "title": "Frigah - HR 32 (79Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Frigah",
+ "coords": {
+ "x": 119.21875,
+ "y": -228.375,
+ "z": 62.5
+ }
+ }
+ },
+ {
+ "title": "Ceuts - HR 32 (60Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Ceuts",
+ "coords": {
+ "x": 110.21875,
+ "y": -214.125,
+ "z": 108.03125
+ }
+ }
+ },
+ {
+ "title": "Vasho - Col 285 Sector YU-F c11-1 (101Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Vasho",
+ "coords": {
+ "x": 125.0625,
+ "y": -169.46875,
+ "z": 157.59375
+ }
+ }
+ },
+ {
+ "title": "Ntumusho - HR 32 (95Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Ntumusho",
+ "coords": {
+ "x": 166.5625,
+ "y": -194.4375,
+ "z": 17.8125
+ }
+ }
+ },
+ {
+ "title": "Hercuna - HR 32 (63Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Hercuna",
+ "coords": {
+ "x": 159.78125,
+ "y": -195.5625,
+ "z": 55.53125
+ }
+ }
+ },
+ {
+ "title": "Inars - Col 285 Sector YU-F c11-1 (90Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Inars",
+ "coords": {
+ "x": 162.9375,
+ "y": -152.625,
+ "z": 115.96875
+ }
+ }
+ },
+ {
+ "title": "Mannovichs - Synuefe BR-L c24-24 (96Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Mannovichs",
+ "coords": {
+ "x": 196.4375,
+ "y": -145.625,
+ "z": 80.03125
+ }
+ }
+ },
+ {
+ "title": "Koko - Col 285 Sector YU-F c11-1 (96Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Koko",
+ "coords": {
+ "x": 98.15625,
+ "y": -164.6875,
+ "z": 160.53125
+ }
+ }
+ },
+ {
+ "title": "Pekoe - Eol Prou LW-L c8-127 (50Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Pekoe",
+ "coords": {
+ "x": -9485.75,
+ "y": -887.96875,
+ "z": 19804.21875
+ }
+ }
+ },
+ {
+ "title": "Poe - Eol Prou LW-L c8-127 (34Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Poe",
+ "coords": {
+ "x": -9496.25,
+ "y": -920.3125,
+ "z": 19803.78125
+ }
+ }
+ },
+ {
+ "title": "Pleiades Sector IH-V c2-16 - Pleiades Sector HR-W d1-79 (12Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Pleiades Sector IH-V c2-16",
+ "coords": {
+ "x": -92.0,
+ "y": -143.5,
+ "z": -341.3125
+ }
+ }
+ },
+ {
+ "title": "Havans - Hyades Sector LC-L b8-0 (124Ly)",
+ "prison": {
+ "name": "Hyades Sector LC-L b8-0",
+ "coords": {
+ "x": 93.71875,
+ "y": -103.625,
+ "z": -85.9375
+ }
+ },
+ "jump": {
+ "name": "Havans",
+ "coords": {
+ "x": 124.9375,
+ "y": -220.40625,
+ "z": -56.625
+ }
+ }
+ },
+ {
+ "title": "Oshatha - HR 32 (62Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Oshatha",
+ "coords": {
+ "x": 128.53125,
+ "y": -220.3125,
+ "z": 86.625
+ }
+ }
+ },
+ {
+ "title": "Pa Kammai - Col 285 Sector YU-F c11-1 (99Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Pa Kammai",
+ "coords": {
+ "x": 95.0625,
+ "y": -161.5625,
+ "z": 168.34375
+ }
+ }
+ },
+ {
+ "title": "Andriani - Col 285 Sector YU-F c11-1 (93Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Andriani",
+ "coords": {
+ "x": 161.90625,
+ "y": -158.84375,
+ "z": 114.59375
+ }
+ }
+ },
+ {
+ "title": "Lisaaka - Synuefe BR-L c24-24 (55Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Lisaaka",
+ "coords": {
+ "x": 202.5625,
+ "y": -173.96875,
+ "z": 12.15625
+ }
+ }
+ },
+ {
+ "title": "Nambi - Capricorni Sector FM-V b2-0 (152Ly)",
+ "prison": {
+ "name": "Capricorni Sector FM-V b2-0",
+ "coords": {
+ "x": -103.59375,
+ "y": -100.375,
+ "z": 97.4375
+ }
+ },
+ "jump": {
+ "name": "Nambi",
+ "coords": {
+ "x": -6.6875,
+ "y": -211.75,
+ "z": 134.875
+ }
+ }
+ },
+ {
+ "title": "Sahamocoria - Col 285 Sector YU-F c11-1 (100Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Sahamocoria",
+ "coords": {
+ "x": 98.25,
+ "y": -164.15625,
+ "z": 167.9375
+ }
+ }
+ },
+ {
+ "title": "Gyhlder - Col 285 Sector YU-F c11-1 (119Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Gyhlder",
+ "coords": {
+ "x": 141.34375,
+ "y": -201.65625,
+ "z": 119.90625
+ }
+ }
+ },
+ {
+ "title": "Tirai - Synuefe BR-L c24-24 (60Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Tirai",
+ "coords": {
+ "x": 201.53125,
+ "y": -182.25,
+ "z": 2.15625
+ }
+ }
+ },
+ {
+ "title": "Olelatha - Synuefe BR-L c24-24 (62Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Olelatha",
+ "coords": {
+ "x": 202.34375,
+ "y": -155.1875,
+ "z": 41.5625
+ }
+ }
+ },
+ {
+ "title": "Uneleni - Col 285 Sector YU-F c11-1 (107Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Uneleni",
+ "coords": {
+ "x": 116.21875,
+ "y": -196.46875,
+ "z": 114.3125
+ }
+ }
+ },
+ {
+ "title": "Umvelli - HR 32 (73Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Umvelli",
+ "coords": {
+ "x": 141.21875,
+ "y": -210.34375,
+ "z": 47.40625
+ }
+ }
+ },
+ {
+ "title": "Anotchi - Col 285 Sector YU-F c11-1 (122Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Anotchi",
+ "coords": {
+ "x": 143.5625,
+ "y": -203.40625,
+ "z": 120.59375
+ }
+ }
+ },
+ {
+ "title": "Gerdia - Synuefe BR-L c24-24 (59Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Gerdia",
+ "coords": {
+ "x": 202.09375,
+ "y": -182.46875,
+ "z": -2.78125
+ }
+ }
+ },
+ {
+ "title": "Abrigi - HR 32 (97Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Abrigi",
+ "coords": {
+ "x": 190.875,
+ "y": -181.84375,
+ "z": 25.625
+ }
+ }
+ },
+ {
+ "title": "Solitude - Eol Prou LW-L c8-127 (31Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Solitude",
+ "coords": {
+ "x": -9497.65625,
+ "y": -911.0,
+ "z": 19807.625
+ }
+ }
+ },
+ {
+ "title": "Hyades Sector AB-W b2-2 - Pleiades Sector HR-W d1-79 (146Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Hyades Sector AB-W b2-2",
+ "coords": {
+ "x": -91.65625,
+ "y": -62.53125,
+ "z": -224.5
+ }
+ }
+ },
+ {
+ "title": "Snake Sector OD-S b4-2 - Alrai Sector KN-S b4-4 (505Ly)",
+ "prison": {
+ "name": "Alrai Sector KN-S b4-4",
+ "coords": {
+ "x": -34.96875,
+ "y": 77.625,
+ "z": 101.59375
+ }
+ },
+ "jump": {
+ "name": "Snake Sector OD-S b4-2",
+ "coords": {
+ "x": -40.375,
+ "y": 63.15625,
+ "z": 605.96875
+ }
+ }
+ },
+ {
+ "title": "Antanoai - ICZ KS-T b3-5 (105Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Antanoai",
+ "coords": {
+ "x": 29.78125,
+ "y": -241.90625,
+ "z": 56.1875
+ }
+ }
+ },
+ {
+ "title": "Jabaja - ICZ KS-T b3-5 (141Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Jabaja",
+ "coords": {
+ "x": 72.03125,
+ "y": -243.5,
+ "z": -22.53125
+ }
+ }
+ },
+ {
+ "title": "Juradhis - Col 285 Sector YU-F c11-1 (136Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Juradhis",
+ "coords": {
+ "x": 61.53125,
+ "y": -221.25,
+ "z": 120.78125
+ }
+ }
+ },
+ {
+ "title": "Selkuntan - Col 285 Sector YU-F c11-1 (152Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Selkuntan",
+ "coords": {
+ "x": 84.09375,
+ "y": -239.09375,
+ "z": 61.1875
+ }
+ }
+ },
+ {
+ "title": "Nicocheo - Col 285 Sector YU-F c11-1 (130Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Nicocheo",
+ "coords": {
+ "x": 75.8125,
+ "y": -220.1875,
+ "z": 107.4375
+ }
+ }
+ },
+ {
+ "title": "Bavapri - HR 32 (115Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Bavapri",
+ "coords": {
+ "x": 111.78125,
+ "y": -240.125,
+ "z": 19.53125
+ }
+ }
+ },
+ {
+ "title": "Monjiwatka - HR 32 (104Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Monjiwatka",
+ "coords": {
+ "x": 96.375,
+ "y": -237.1875,
+ "z": 40.84375
+ }
+ }
+ },
+ {
+ "title": "Beara - Col 285 Sector YU-F c11-1 (150Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Beara",
+ "coords": {
+ "x": 97.09375,
+ "y": -238.78125,
+ "z": 63.46875
+ }
+ }
+ },
+ {
+ "title": "Wadjirusi - HR 32 (77Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Wadjirusi",
+ "coords": {
+ "x": 100.5625,
+ "y": -225.78125,
+ "z": 77.375
+ }
+ }
+ },
+ {
+ "title": "Wanano - HR 32 (77Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Wanano",
+ "coords": {
+ "x": 97.53125,
+ "y": -224.6875,
+ "z": 78.375
+ }
+ }
+ },
+ {
+ "title": "He Qinga - HR 32 (148Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "He Qinga",
+ "coords": {
+ "x": 129.34375,
+ "y": -240.34375,
+ "z": -24.125
+ }
+ }
+ },
+ {
+ "title": "Dangkhurs - HR 32 (137Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Dangkhurs",
+ "coords": {
+ "x": 118.34375,
+ "y": -222.84375,
+ "z": -21.125
+ }
+ }
+ },
+ {
+ "title": "Apsaras - HR 32 (74Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Apsaras",
+ "coords": {
+ "x": 130.28125,
+ "y": -223.9375,
+ "z": 64.34375
+ }
+ }
+ },
+ {
+ "title": "Edinura - Col 285 Sector YU-F c11-1 (102Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Edinura",
+ "coords": {
+ "x": 128.53125,
+ "y": -189.5625,
+ "z": 100.96875
+ }
+ }
+ },
+ {
+ "title": "Ac Yaming - Col 285 Sector YU-F c11-1 (118Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Ac Yaming",
+ "coords": {
+ "x": 133.4375,
+ "y": -200.375,
+ "z": 131.875
+ }
+ }
+ },
+ {
+ "title": "Khonti - Synuefe BR-L c24-24 (109Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Khonti",
+ "coords": {
+ "x": 150.84375,
+ "y": -206.84375,
+ "z": -12.46875
+ }
+ }
+ },
+ {
+ "title": "Vaftetia - Col 285 Sector YU-F c11-1 (114Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Vaftetia",
+ "coords": {
+ "x": 141.71875,
+ "y": -195.1875,
+ "z": 75.0625
+ }
+ }
+ },
+ {
+ "title": "Estarna - Col 285 Sector YU-F c11-1 (114Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Estarna",
+ "coords": {
+ "x": 148.84375,
+ "y": -194.28125,
+ "z": 106.375
+ }
+ }
+ },
+ {
+ "title": "Songo - HR 32 (91Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Songo",
+ "coords": {
+ "x": 160.5,
+ "y": -206.3125,
+ "z": 26.90625
+ }
+ }
+ },
+ {
+ "title": "Liu Xuan De - HR 32 (79Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Liu Xuan De",
+ "coords": {
+ "x": 173.90625,
+ "y": -188.1875,
+ "z": 39.03125
+ }
+ }
+ },
+ {
+ "title": "Alourovices - HR 32 (49Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Alourovices",
+ "coords": {
+ "x": 172.53125,
+ "y": -184.125,
+ "z": 87.03125
+ }
+ }
+ },
+ {
+ "title": "Yulashis - HR 32 (90Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Yulashis",
+ "coords": {
+ "x": 190.0625,
+ "y": -170.40625,
+ "z": 30.4375
+ }
+ }
+ },
+ {
+ "title": "Pinggul - Synuefe BR-L c24-24 (133Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Pinggul",
+ "coords": {
+ "x": 145.34375,
+ "y": -215.0625,
+ "z": 50.25
+ }
+ }
+ },
+ {
+ "title": "Almasci - HR 32 (105Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Almasci",
+ "coords": {
+ "x": 200.28125,
+ "y": -177.15625,
+ "z": 21.53125
+ }
+ }
+ },
+ {
+ "title": "Droju OH-T a99-0 - Omega Sector OD-S b4-0 (1871Ly)",
+ "prison": {
+ "name": "Omega Sector OD-S b4-0",
+ "coords": {
+ "x": -1456.8125,
+ "y": -84.8125,
+ "z": 5306.9375
+ }
+ },
+ "jump": {
+ "name": "Droju OH-T a99-0",
+ "coords": {
+ "x": -297.625,
+ "y": -26.59375,
+ "z": 3839.40625
+ }
+ }
+ },
+ {
+ "title": "Yakamutii - Col 285 Sector YU-F c11-1 (104Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Yakamutii",
+ "coords": {
+ "x": 141.375,
+ "y": -183.65625,
+ "z": 122.46875
+ }
+ }
+ },
+ {
+ "title": "Angardh - Synuefe BR-L c24-24 (95Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Angardh",
+ "coords": {
+ "x": 178.90625,
+ "y": -165.90625,
+ "z": 64.375
+ }
+ }
+ },
+ {
+ "title": "Wardh - HR 32 (102Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Wardh",
+ "coords": {
+ "x": 205.375,
+ "y": -173.1875,
+ "z": 28.75
+ }
+ }
+ },
+ {
+ "title": "Xuangeriva - HR 32 (87Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Xuangeriva",
+ "coords": {
+ "x": 212.40625,
+ "y": -136.625,
+ "z": 73.8125
+ }
+ }
+ },
+ {
+ "title": "Bleia Dryiae EE-E d13-178 - Omega Sector OD-S b4-0 (470Ly)",
+ "prison": {
+ "name": "Omega Sector OD-S b4-0",
+ "coords": {
+ "x": -1456.8125,
+ "y": -84.8125,
+ "z": 5306.9375
+ }
+ },
+ "jump": {
+ "name": "Bleia Dryiae EE-E d13-178",
+ "coords": {
+ "x": -992.84375,
+ "y": -49.78125,
+ "z": 5243.25
+ }
+ }
+ },
+ {
+ "title": "Sadr Region Sector GW-W c1-22 - Capricorni Sector FM-V b2-0 (1717Ly)",
+ "prison": {
+ "name": "Capricorni Sector FM-V b2-0",
+ "coords": {
+ "x": -103.59375,
+ "y": -100.375,
+ "z": 97.4375
+ }
+ },
+ "jump": {
+ "name": "Sadr Region Sector GW-W c1-22",
+ "coords": {
+ "x": -1792.125,
+ "y": 52.65625,
+ "z": 369.5625
+ }
+ }
+ },
+ {
+ "title": "T'ien - Col 285 Sector YU-F c11-1 (95Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "T'ien",
+ "coords": {
+ "x": 136.75,
+ "y": -178.46875,
+ "z": 113.28125
+ }
+ }
+ },
+ {
+ "title": "Fuambe - HR 32 (85Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Fuambe",
+ "coords": {
+ "x": 205.28125,
+ "y": -182.9375,
+ "z": 61.5625
+ }
+ }
+ },
+ {
+ "title": "Sugagarr - Col 285 Sector YU-F c11-1 (111Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Sugagarr",
+ "coords": {
+ "x": 100.3125,
+ "y": -180.25,
+ "z": 166.28125
+ }
+ }
+ },
+ {
+ "title": "Upem - HR 32 (94Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Upem",
+ "coords": {
+ "x": 207.96875,
+ "y": -140.84375,
+ "z": 46.0625
+ }
+ }
+ },
+ {
+ "title": "Musca Dark Region IM-V c2-24 - Crucis Sector ND-S b4-5 (437Ly)",
+ "prison": {
+ "name": "Crucis Sector ND-S b4-5",
+ "coords": {
+ "x": 78.6875,
+ "y": 53.9375,
+ "z": 80.03125
+ }
+ },
+ "jump": {
+ "name": "Musca Dark Region IM-V c2-24",
+ "coords": {
+ "x": 458.25,
+ "y": -8.8125,
+ "z": 287.09375
+ }
+ }
+ },
+ {
+ "title": "Phoenix - Eol Prou LW-L c8-127 (27Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Phoenix",
+ "coords": {
+ "x": -9544.21875,
+ "y": -906.84375,
+ "z": 19830.28125
+ }
+ }
+ },
+ {
+ "title": "Omana - HR 32 (89Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Omana",
+ "coords": {
+ "x": 210.34375,
+ "y": -116.96875,
+ "z": 88.4375
+ }
+ }
+ },
+ {
+ "title": "Prua Phoe PI-I b55-3 - Stuelou AT-J c25-24 (2576Ly)",
+ "prison": {
+ "name": "Stuelou AT-J c25-24",
+ "coords": {
+ "x": -6704.4375,
+ "y": -156.34375,
+ "z": 14101.875
+ }
+ },
+ "jump": {
+ "name": "Prua Phoe PI-I b55-3",
+ "coords": {
+ "x": -5994.28125,
+ "y": -413.4375,
+ "z": 11638.9375
+ }
+ }
+ },
+ {
+ "title": "Ekonii - ICZ KS-T b3-5 (105Ly)",
+ "prison": {
+ "name": "ICZ KS-T b3-5",
+ "coords": {
+ "x": -2.84375,
+ "y": -143.3125,
+ "z": 42.75
+ }
+ },
+ "jump": {
+ "name": "Ekonii",
+ "coords": {
+ "x": 28.625,
+ "y": -236.71875,
+ "z": 79.03125
+ }
+ }
+ },
+ {
+ "title": "Khodur - HR 32 (115Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Khodur",
+ "coords": {
+ "x": 62.8125,
+ "y": -244.03125,
+ "z": 65.09375
+ }
+ }
+ },
+ {
+ "title": "Kunbure - Col 285 Sector YU-F c11-1 (135Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Kunbure",
+ "coords": {
+ "x": 66.8125,
+ "y": -222.03125,
+ "z": 119.75
+ }
+ }
+ },
+ {
+ "title": "Shui - HR 32 (140Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Shui",
+ "coords": {
+ "x": 88.1875,
+ "y": -237.6875,
+ "z": -8.0625
+ }
+ }
+ },
+ {
+ "title": "Ixtabru - HR 32 (145Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Ixtabru",
+ "coords": {
+ "x": 124.28125,
+ "y": -239.625,
+ "z": -21.0
+ }
+ }
+ },
+ {
+ "title": "Han Tsanyan - HR 32 (103Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Han Tsanyan",
+ "coords": {
+ "x": 129.9375,
+ "y": -235.6875,
+ "z": 29.90625
+ }
+ }
+ },
+ {
+ "title": "Zac Matlan - HR 32 (89Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Zac Matlan",
+ "coords": {
+ "x": 122.46875,
+ "y": -234.0,
+ "z": 51.75
+ }
+ }
+ },
+ {
+ "title": "Banji - HR 32 (82Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Banji",
+ "coords": {
+ "x": 134.21875,
+ "y": -240.34375,
+ "z": 85.125
+ }
+ }
+ },
+ {
+ "title": "Balanek - HR 32 (76Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Balanek",
+ "coords": {
+ "x": 118.71875,
+ "y": -216.40625,
+ "z": 51.0625
+ }
+ }
+ },
+ {
+ "title": "Koya Gu - HR 32 (73Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Koya Gu",
+ "coords": {
+ "x": 134.53125,
+ "y": -225.0,
+ "z": 68.28125
+ }
+ }
+ },
+ {
+ "title": "Samo - Col 285 Sector YU-F c11-1 (102Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Samo",
+ "coords": {
+ "x": 133.28125,
+ "y": -187.53125,
+ "z": 113.6875
+ }
+ }
+ },
+ {
+ "title": "Narveti - HR 32 (120Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Narveti",
+ "coords": {
+ "x": 153.1875,
+ "y": -206.1875,
+ "z": -9.5
+ }
+ }
+ },
+ {
+ "title": "Gaezani - Synuefe BR-L c24-24 (130Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Gaezani",
+ "coords": {
+ "x": 142.15625,
+ "y": -216.34375,
+ "z": 35.65625
+ }
+ }
+ },
+ {
+ "title": "Blandagur - Col 285 Sector YU-F c11-1 (127Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Blandagur",
+ "coords": {
+ "x": 138.46875,
+ "y": -211.21875,
+ "z": 73.4375
+ }
+ }
+ },
+ {
+ "title": "Caspiat - Col 285 Sector YU-F c11-1 (134Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Caspiat",
+ "coords": {
+ "x": 154.0625,
+ "y": -212.59375,
+ "z": 75.34375
+ }
+ }
+ },
+ {
+ "title": "Ten Mayan - Col 285 Sector YU-F c11-1 (116Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Ten Mayan",
+ "coords": {
+ "x": 143.9375,
+ "y": -198.78125,
+ "z": 105.8125
+ }
+ }
+ },
+ {
+ "title": "Har Pa Kams - Synuefe BR-L c24-24 (89Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Har Pa Kams",
+ "coords": {
+ "x": 165.6875,
+ "y": -192.8125,
+ "z": 2.5625
+ }
+ }
+ },
+ {
+ "title": "Basuseku - HR 32 (71Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Basuseku",
+ "coords": {
+ "x": 158.34375,
+ "y": -201.96875,
+ "z": 49.125
+ }
+ }
+ },
+ {
+ "title": "Votadja - Col 285 Sector YU-F c11-1 (114Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Votadja",
+ "coords": {
+ "x": 165.6875,
+ "y": -184.90625,
+ "z": 90.28125
+ }
+ }
+ },
+ {
+ "title": "Bhilonggam - HR 32 (91Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Bhilonggam",
+ "coords": {
+ "x": 191.6875,
+ "y": -167.9375,
+ "z": 30.40625
+ }
+ }
+ },
+ {
+ "title": "Lycanthrope - Eol Prou LW-L c8-127 (41Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Lycanthrope",
+ "coords": {
+ "x": -9506.4375,
+ "y": -944.125,
+ "z": 19795.0625
+ }
+ }
+ },
+ {
+ "title": "Trifid Sector GW-W d1-220 - Omega Sector OD-S b4-0 (849Ly)",
+ "prison": {
+ "name": "Omega Sector OD-S b4-0",
+ "coords": {
+ "x": -1456.8125,
+ "y": -84.8125,
+ "z": 5306.9375
+ }
+ },
+ "jump": {
+ "name": "Trifid Sector GW-W d1-220",
+ "coords": {
+ "x": -618.71875,
+ "y": -24.90625,
+ "z": 5184.34375
+ }
+ }
+ },
+ {
+ "title": "Hephaestus - Eol Prou LW-L c8-127 (12Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Hephaestus",
+ "coords": {
+ "x": -9521.40625,
+ "y": -907.3125,
+ "z": 19801.65625
+ }
+ }
+ },
+ {
+ "title": "Plaa Aescs QD-T c3-28 - Nyeajaae VU-Y a27-9 (855Ly)",
+ "prison": {
+ "name": "Nyeajaae VU-Y a27-9",
+ "coords": {
+ "x": -3374,
+ "y": -42.375,
+ "z": 6907.96875
+ }
+ },
+ "jump": {
+ "name": "Plaa Aescs QD-T c3-28",
+ "coords": {
+ "x": -2530.78125,
+ "y": 45.21875,
+ "z": 6793.40625
+ }
+ }
+ },
+ {
+ "title": "NGC 6188 Sector LC-V c2-28 - Omega Sector OD-S b4-0 (3401Ly)",
+ "prison": {
+ "name": "Omega Sector OD-S b4-0",
+ "coords": {
+ "x": -1456.8125,
+ "y": -84.8125,
+ "z": 5306.9375
+ }
+ },
+ "jump": {
+ "name": "NGC 6188 Sector LC-V c2-28",
+ "coords": {
+ "x": 1706.1875,
+ "y": -87.625,
+ "z": 4057.15625
+ }
+ }
+ },
+ {
+ "title": "Wregoe XQ-L c21-29 - Synuefe BR-L c24-24 (269Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Wregoe XQ-L c21-29",
+ "coords": {
+ "x": 378.53125,
+ "y": 48.4375,
+ "z": -143.1875
+ }
+ }
+ },
+ {
+ "title": "Canis Subridens - Eol Prou LW-L c8-127 (37Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Canis Subridens",
+ "coords": {
+ "x": -9502.125,
+ "y": -921.3125,
+ "z": 19784.96875
+ }
+ }
+ },
+ {
+ "title": "Mriya - Eol Prou LW-L c8-127 (26Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Mriya",
+ "coords": {
+ "x": -9515.4375,
+ "y": -905.75,
+ "z": 19830.8125
+ }
+ }
+ },
+ {
+ "title": "Blua Eaec US-Z b46-4 - Stuelou AT-J c25-24 (1287Ly)",
+ "prison": {
+ "name": "Stuelou AT-J c25-24",
+ "coords": {
+ "x": -6704.4375,
+ "y": -156.34375,
+ "z": 14101.875
+ }
+ },
+ "jump": {
+ "name": "Blua Eaec US-Z b46-4",
+ "coords": {
+ "x": -7173.71875,
+ "y": -261.625,
+ "z": 15295.40625
+ }
+ }
+ },
+ {
+ "title": "Byeia Eurk IE-L b49-4 - Omega Sector OD-S b4-0 (1196Ly)",
+ "prison": {
+ "name": "Omega Sector OD-S b4-0",
+ "coords": {
+ "x": -1456.8125,
+ "y": -84.8125,
+ "z": 5306.9375
+ }
+ },
+ "jump": {
+ "name": "Byeia Eurk IE-L b49-4",
+ "coords": {
+ "x": -1898.875,
+ "y": 37.28125,
+ "z": 6411.03125
+ }
+ }
+ },
+ {
+ "title": "Robigo - Pleiades Sector HR-W d1-79 (272Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Robigo",
+ "coords": {
+ "x": -303.40625,
+ "y": 7.3125,
+ "z": -314.15625
+ }
+ }
+ },
+ {
+ "title": "Pleiades Sector AB-W b2-4 - Pleiades Sector HR-W d1-79 (74Ly)",
+ "prison": {
+ "name": "Pleiades Sector HR-W d1-79",
+ "coords": {
+ "x": -80.625,
+ "y": -146.65625,
+ "z": -343.25
+ }
+ },
+ "jump": {
+ "name": "Pleiades Sector AB-W b2-4",
+ "coords": {
+ "x": -137.5625,
+ "y": -118.25,
+ "z": -380.4375
+ }
+ }
+ },
+ {
+ "title": "Khary - Col 285 Sector YU-F c11-1 (149Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Khary",
+ "coords": {
+ "x": 93.96875,
+ "y": -241.78125,
+ "z": 93.96875
+ }
+ }
+ },
+ {
+ "title": "Waimile - Col 285 Sector YU-F c11-1 (116Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Waimile",
+ "coords": {
+ "x": 128.5,
+ "y": -202.40625,
+ "z": 120.71875
+ }
+ }
+ },
+ {
+ "title": "Sental - Synuefe BR-L c24-24 (124Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Sental",
+ "coords": {
+ "x": 142.65625,
+ "y": -217.59375,
+ "z": 7.375
+ }
+ }
+ },
+ {
+ "title": "Chonta - HR 32 (68Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Chonta",
+ "coords": {
+ "x": 151.90625,
+ "y": -200.59375,
+ "z": 48.1875
+ }
+ }
+ },
+ {
+ "title": "Jamab - Col 285 Sector YU-F c11-1 (112Ly)",
+ "prison": {
+ "name": "Col 285 Sector YU-F c11-1",
+ "coords": {
+ "x": 98.53125,
+ "y": -92.625,
+ "z": 97.625
+ }
+ },
+ "jump": {
+ "name": "Jamab",
+ "coords": {
+ "x": 139.75,
+ "y": -197.0,
+ "z": 99.28125
+ }
+ }
+ },
+ {
+ "title": "Mukhang - HR 32 (74Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Mukhang",
+ "coords": {
+ "x": 162.46875,
+ "y": -200.25,
+ "z": 44.96875
+ }
+ }
+ },
+ {
+ "title": "Kikab - HR 32 (40Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Kikab",
+ "coords": {
+ "x": 163.5,
+ "y": -181.5,
+ "z": 87.40625
+ }
+ }
+ },
+ {
+ "title": "Oicorio - Synuefe BR-L c24-24 (90Ly)",
+ "prison": {
+ "name": "Synuefe BR-L c24-24",
+ "coords": {
+ "x": 228.375,
+ "y": -130.09375,
+ "z": -8.8125
+ }
+ },
+ "jump": {
+ "name": "Oicorio",
+ "coords": {
+ "x": 178.96875,
+ "y": -180.15625,
+ "z": 47.875
+ }
+ }
+ },
+ {
+ "title": "Meretrida - Eol Prou LW-L c8-127 (28Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Meretrida",
+ "coords": {
+ "x": -9515.71875,
+ "y": -899.625,
+ "z": 19830.3125
+ }
+ }
+ },
+ {
+ "title": "Santos Dumont - Eol Prou LW-L c8-127 (34Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Santos Dumont",
+ "coords": {
+ "x": -9549.53125,
+ "y": -910.34375,
+ "z": 19836.65625
+ }
+ }
+ },
+ {
+ "title": "Ra' Takakhan - Eol Prou LW-L c8-127 (32Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Ra' Takakhan",
+ "coords": {
+ "x": -9530.375,
+ "y": -911.0,
+ "z": 19840.9375
+ }
+ }
+ },
+ {
+ "title": "Pyrrha - Eol Prou LW-L c8-127 (20Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Pyrrha",
+ "coords": {
+ "x": -9529.59375,
+ "y": -902.03125,
+ "z": 19826.375
+ }
+ }
+ },
+ {
+ "title": "Trakath - Eol Prou LW-L c8-127 (13Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Trakath",
+ "coords": {
+ "x": -9534.78125,
+ "y": -908.96875,
+ "z": 19798.9375
+ }
+ }
+ },
+ {
+ "title": "Sollaro - Eol Prou LW-L c8-127 (28Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Sollaro",
+ "coords": {
+ "x": -9528.625,
+ "y": -885.59375,
+ "z": 19815.4375
+ }
+ }
+ },
+ {
+ "title": "Carlota - Eol Prou LW-L c8-127 (40Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Carlota",
+ "coords": {
+ "x": -9531.40625,
+ "y": -874.96875,
+ "z": 19797.53125
+ }
+ }
+ },
+ {
+ "title": "Union - Eol Prou LW-L c8-127 (33Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Union",
+ "coords": {
+ "x": -9540.71875,
+ "y": -882.9375,
+ "z": 19810.09375
+ }
+ }
+ },
+ {
+ "title": "Prua Phoe MI-B b17-5 - Stuelou AT-J c25-24 (3474Ly)",
+ "prison": {
+ "name": "Stuelou AT-J c25-24",
+ "coords": {
+ "x": -6704.4375,
+ "y": -156.34375,
+ "z": 14101.875
+ }
+ },
+ "jump": {
+ "name": "Prua Phoe MI-B b17-5",
+ "coords": {
+ "x": -5652.84375,
+ "y": -561.0625,
+ "z": 10815.34375
+ }
+ }
+ },
+ {
+ "title": "Skaudai GM-S b35-5 - Nyeajaae VU-Y a27-9 (3587Ly)",
+ "prison": {
+ "name": "Nyeajaae VU-Y a27-9",
+ "coords": {
+ "x": -3374,
+ "y": -42.375,
+ "z": 6907.96875
+ }
+ },
+ "jump": {
+ "name": "Skaudai GM-S b35-5",
+ "coords": {
+ "x": -5221.1875,
+ "y": -525.0,
+ "z": 9945.03125
+ }
+ }
+ },
+ {
+ "title": "Hemait - HR 32 (70Ly)",
+ "prison": {
+ "name": "HR 32",
+ "coords": {
+ "x": 132.5,
+ "y": -159.25,
+ "z": 99.1875
+ }
+ },
+ "jump": {
+ "name": "Hemait",
+ "coords": {
+ "x": 164.21875,
+ "y": -196.28125,
+ "z": 49.53125
+ }
+ }
+ },
+ {
+ "title": "Los - Eol Prou LW-L c8-127 (35Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Los",
+ "coords": {
+ "x": -9509.34375,
+ "y": -886.3125,
+ "z": 19820.125
+ }
+ }
+ },
+ {
+ "title": "Pergamon - Eol Prou LW-L c8-127 (18Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Pergamon",
+ "coords": {
+ "x": -9520.9375,
+ "y": -896.65625,
+ "z": 19808.78125
+ }
+ }
+ },
+ {
+ "title": "Asura - Eol Prou LW-L c8-127 (23Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Asura",
+ "coords": {
+ "x": -9550.28125,
+ "y": -916.65625,
+ "z": 19816.1875
+ }
+ }
+ },
+ {
+ "title": "Kajuku - Eol Prou LW-L c8-127 (33Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Kajuku",
+ "coords": {
+ "x": -9525.03125,
+ "y": -943.40625,
+ "z": 19795.5625
+ }
+ }
+ },
+ {
+ "title": "Valac - Eol Prou LW-L c8-127 (26Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Valac",
+ "coords": {
+ "x": -9535.6875,
+ "y": -914.59375,
+ "z": 19784.78125
+ }
+ }
+ },
+ {
+ "title": "Canonnia - Eol Prou LW-L c8-127 (27Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Canonnia",
+ "coords": {
+ "x": -9522.9375,
+ "y": -894.0625,
+ "z": 19791.875
+ }
+ }
+ },
+ {
+ "title": "Einheriar - Eol Prou LW-L c8-127 (45Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Einheriar",
+ "coords": {
+ "x": -9557.8125,
+ "y": -880.1875,
+ "z": 19801.5625
+ }
+ }
+ },
+ {
+ "title": "Saraswati - Eol Prou LW-L c8-127 (20Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Saraswati",
+ "coords": {
+ "x": -9538.0,
+ "y": -896.40625,
+ "z": 19804.71875
+ }
+ }
+ },
+ {
+ "title": "Flyiedge KP-K b27-6 - Nyeajaae VU-Y a27-9 (1798Ly)",
+ "prison": {
+ "name": "Nyeajaae VU-Y a27-9",
+ "coords": {
+ "x": -3374,
+ "y": -42.375,
+ "z": 6907.96875
+ }
+ },
+ "jump": {
+ "name": "Flyiedge KP-K b27-6",
+ "coords": {
+ "x": -4209.59375,
+ "y": -266.21875,
+ "z": 8484.09375
+ }
+ }
+ },
+ {
+ "title": "Coalsack Sector VU-O b6-6 - Crucis Sector ND-S b4-5 (409Ly)",
+ "prison": {
+ "name": "Crucis Sector ND-S b4-5",
+ "coords": {
+ "x": 78.6875,
+ "y": 53.9375,
+ "z": 80.03125
+ }
+ },
+ "jump": {
+ "name": "Coalsack Sector VU-O b6-6",
+ "coords": {
+ "x": 418.65625,
+ "y": 24.25,
+ "z": 304.5625
+ }
+ }
+ },
+ {
+ "title": "Edge Fraternity Landing - Eol Prou LW-L c8-127 (28Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Edge Fraternity Landing",
+ "coords": {
+ "x": -9544.5625,
+ "y": -889.75,
+ "z": 19810.5
+ }
+ }
+ },
+ {
+ "title": "Magellan - Eol Prou LW-L c8-127 (22Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Magellan",
+ "coords": {
+ "x": -9509.3125,
+ "y": -914.625,
+ "z": 19819.96875
+ }
+ }
+ },
+ {
+ "title": "Flyiedge VN-W c4-51 - Nyeajaae VU-Y a27-9 (1344Ly)",
+ "prison": {
+ "name": "Nyeajaae VU-Y a27-9",
+ "coords": {
+ "x": -3374,
+ "y": -42.375,
+ "z": 6907.96875
+ }
+ },
+ "jump": {
+ "name": "Flyiedge VN-W c4-51",
+ "coords": {
+ "x": -3944.3125,
+ "y": -160.875,
+ "z": 8119.59375
+ }
+ }
+ },
+ {
+ "title": "Mobia - Eol Prou LW-L c8-127 (29Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Mobia",
+ "coords": {
+ "x": -9530.6875,
+ "y": -909.84375,
+ "z": 19780.3125
+ }
+ }
+ },
+ {
+ "title": "Metztli - Eol Prou LW-L c8-127 (18Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Metztli",
+ "coords": {
+ "x": -9518.40625,
+ "y": -911.96875,
+ "z": 19823.625
+ }
+ }
+ },
+ {
+ "title": "Nyeajaae SC-B b33-7 - Nyeajaae VU-Y a27-9 (464Ly)",
+ "prison": {
+ "name": "Nyeajaae VU-Y a27-9",
+ "coords": {
+ "x": -3374,
+ "y": -42.375,
+ "z": 6907.96875
+ }
+ },
+ "jump": {
+ "name": "Nyeajaae SC-B b33-7",
+ "coords": {
+ "x": -3539.8125,
+ "y": -147.09375,
+ "z": 7328.21875
+ }
+ }
+ },
+ {
+ "title": "Lux Caeli - Eol Prou LW-L c8-127 (40Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Lux Caeli",
+ "coords": {
+ "x": -9489.96875,
+ "y": -911.71875,
+ "z": 19818.03125
+ }
+ }
+ },
+ {
+ "title": "Pru Euq WO-D b53-8 - Capricorni Sector FM-V b2-0 (1278Ly)",
+ "prison": {
+ "name": "Capricorni Sector FM-V b2-0",
+ "coords": {
+ "x": -103.59375,
+ "y": -100.375,
+ "z": 97.4375
+ }
+ },
+ "jump": {
+ "name": "Pru Euq WO-D b53-8",
+ "coords": {
+ "x": -256.34375,
+ "y": -5.15625,
+ "z": 1363.125
+ }
+ }
+ },
+ {
+ "title": "Flyiedge JE-Z b46-9 - Nyeajaae VU-Y a27-9 (2280Ly)",
+ "prison": {
+ "name": "Nyeajaae VU-Y a27-9",
+ "coords": {
+ "x": -3374,
+ "y": -42.375,
+ "z": 6907.96875
+ }
+ },
+ "jump": {
+ "name": "Flyiedge JE-Z b46-9",
+ "coords": {
+ "x": -4442.21875,
+ "y": -324.03125,
+ "z": 8901.9375
+ }
+ }
+ },
+ {
+ "title": "Coalsack Sector KN-S b4-9 - Hydrae Sector ON-T b3-1 (362Ly)",
+ "prison": {
+ "name": "Hydrae Sector ON-T b3-1",
+ "coords": {
+ "x": 99.875,
+ "y": 92.96875,
+ "z": 78.0625
+ }
+ },
+ "jump": {
+ "name": "Coalsack Sector KN-S b4-9",
+ "coords": {
+ "x": 405.46875,
+ "y": 40.0,
+ "z": 264.0625
+ }
+ }
+ },
+ {
+ "title": "Ratraii - Eol Prou LW-L c8-127 (8Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Ratraii",
+ "coords": {
+ "x": -9530.96875,
+ "y": -918.03125,
+ "z": 19803.8125
+ }
+ }
+ },
+ {
+ "title": "Ravenhold - Eol Prou LW-L c8-127 (33Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Ravenhold",
+ "coords": {
+ "x": -9548.46875,
+ "y": -938.625,
+ "z": 19801.8125
+ }
+ }
+ },
+ {
+ "title": "Benzaiten - Eol Prou LW-L c8-127 (32Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Benzaiten",
+ "coords": {
+ "x": -9523.15625,
+ "y": -881.875,
+ "z": 19811.3125
+ }
+ }
+ },
+ {
+ "title": "Dryooe Flyou WB-T b47-10 - Eol Prou LW-L c8-127 (705Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Dryooe Flyou WB-T b47-10",
+ "coords": {
+ "x": -9262.09375,
+ "y": -768.59375,
+ "z": 19172.96875
+ }
+ }
+ },
+ {
+ "title": "Lagoon Sector NI-S b4-10 - Omega Sector OD-S b4-0 (1304Ly)",
+ "prison": {
+ "name": "Omega Sector OD-S b4-0",
+ "coords": {
+ "x": -1456.8125,
+ "y": -84.8125,
+ "z": 5306.9375
+ }
+ },
+ "jump": {
+ "name": "Lagoon Sector NI-S b4-10",
+ "coords": {
+ "x": -469.1875,
+ "y": -84.84375,
+ "z": 4456.125
+ }
+ }
+ },
+ {
+ "title": "Rodentia - Eol Prou LW-L c8-127 (23Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Rodentia",
+ "coords": {
+ "x": -9530.53125,
+ "y": -907.25,
+ "z": 19787.375
+ }
+ }
+ },
+ {
+ "title": "Eol Prou PC-K c9-91 - Eol Prou LW-L c8-127 (11Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Eol Prou PC-K c9-91",
+ "coords": {
+ "x": -9533.46875,
+ "y": -913.125,
+ "z": 19819.71875
+ }
+ }
+ },
+ {
+ "title": "Byeia Eurk OC-I b37-13 - Omega Sector OD-S b4-0 (899Ly)",
+ "prison": {
+ "name": "Omega Sector OD-S b4-0",
+ "coords": {
+ "x": -1456.8125,
+ "y": -84.8125,
+ "z": 5306.9375
+ }
+ },
+ "jump": {
+ "name": "Byeia Eurk OC-I b37-13",
+ "coords": {
+ "x": -1781.75,
+ "y": 39.78125,
+ "z": 6136.375
+ }
+ }
+ },
+ {
+ "title": "Chrysus - Eol Prou LW-L c8-127 (17Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Chrysus",
+ "coords": {
+ "x": -9537.9375,
+ "y": -922.1875,
+ "z": 19820.1875
+ }
+ }
+ },
+ {
+ "title": "Nyeajaae NB-Q b52-14 - Nyeajaae VU-Y a27-9 (926Ly)",
+ "prison": {
+ "name": "Nyeajaae VU-Y a27-9",
+ "coords": {
+ "x": -3374,
+ "y": -42.375,
+ "z": 6907.96875
+ }
+ },
+ "jump": {
+ "name": "Nyeajaae NB-Q b52-14",
+ "coords": {
+ "x": -3759.75,
+ "y": -159.25,
+ "z": 7741.15625
+ }
+ }
+ },
+ {
+ "title": "Omega Sector VE-Q b5-15 - Omega Sector OD-S b4-0 (18Ly)",
+ "prison": {
+ "name": "Omega Sector OD-S b4-0",
+ "coords": {
+ "x": -1456.8125,
+ "y": -84.8125,
+ "z": 5306.9375
+ }
+ },
+ "jump": {
+ "name": "Omega Sector VE-Q b5-15",
+ "coords": {
+ "x": -1444.3125,
+ "y": -85.8125,
+ "z": 5319.9375
+ }
+ }
+ },
+ {
+ "title": "Eoch Flyuae ZU-Y b17-16 - Eol Prou LW-L c8-127 (2907Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Eoch Flyuae ZU-Y b17-16",
+ "coords": {
+ "x": -8194.90625,
+ "y": -639.28125,
+ "z": 17240.65625
+ }
+ }
+ },
+ {
+ "title": "Sovereign's Reach - Eol Prou LW-L c8-127 (10Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Sovereign's Reach",
+ "coords": {
+ "x": -9535.96875,
+ "y": -913.78125,
+ "z": 19816.96875
+ }
+ }
+ },
+ {
+ "title": "Prua Phoe VF-M d8-1046 - Stuelou AT-J c25-24 (3046Ly)",
+ "prison": {
+ "name": "Stuelou AT-J c25-24",
+ "coords": {
+ "x": -6704.4375,
+ "y": -156.34375,
+ "z": 14101.875
+ }
+ },
+ "jump": {
+ "name": "Prua Phoe VF-M d8-1046",
+ "coords": {
+ "x": -5858.65625,
+ "y": -396.6875,
+ "z": 11185.0625
+ }
+ }
+ },
+ {
+ "title": "Pennsylvania - Eol Prou LW-L c8-127 (15Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Pennsylvania",
+ "coords": {
+ "x": -9513.9375,
+ "y": -911.34375,
+ "z": 19809.90625
+ }
+ }
+ },
+ {
+ "title": "Desy - Eol Prou LW-L c8-127 (18Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Desy",
+ "coords": {
+ "x": -9534.21875,
+ "y": -912.21875,
+ "z": 19792.375
+ }
+ }
+ },
+ {
+ "title": "Eoch Flyuae PL-D c138 - Stuelou AT-J c25-24 (3091Ly)",
+ "prison": {
+ "name": "Stuelou AT-J c25-24",
+ "coords": {
+ "x": -6704.4375,
+ "y": -156.34375,
+ "z": 14101.875
+ }
+ },
+ "jump": {
+ "name": "Eoch Flyuae PL-D c138",
+ "coords": {
+ "x": -7985.1875,
+ "y": -561.5625,
+ "z": 16886.21875
+ }
+ }
+ },
+ {
+ "title": "Kinesi - Eol Prou LW-L c8-127 (27Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Kinesi",
+ "coords": {
+ "x": -9510.3125,
+ "y": -895.53125,
+ "z": 19799.875
+ }
+ }
+ },
+ {
+ "title": "Pytheas - Eol Prou LW-L c8-127 (17Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Pytheas",
+ "coords": {
+ "x": -9524.53125,
+ "y": -909.0625,
+ "z": 19793.28125
+ }
+ }
+ },
+ {
+ "title": "Blua Eaec RD-Z d1-1228 - Stuelou AT-J c25-24 (457Ly)",
+ "prison": {
+ "name": "Stuelou AT-J c25-24",
+ "coords": {
+ "x": -6704.4375,
+ "y": -156.34375,
+ "z": 14101.875
+ }
+ },
+ "jump": {
+ "name": "Blua Eaec RD-Z d1-1228",
+ "coords": {
+ "x": -6857.40625,
+ "y": -159.78125,
+ "z": 14532.125
+ }
+ }
+ },
+ {
+ "title": "Kopernik - Eol Prou LW-L c8-127 (28Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Kopernik",
+ "coords": {
+ "x": -9504.75,
+ "y": -901.75,
+ "z": 19801.875
+ }
+ }
+ },
+ {
+ "title": "Carcosa - Eol Prou LW-L c8-127 (39Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Carcosa",
+ "coords": {
+ "x": -9544.25,
+ "y": -879.59375,
+ "z": 19822.03125
+ }
+ }
+ },
+ {
+ "title": "Garuda - Eol Prou LW-L c8-127 (25Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Garuda",
+ "coords": {
+ "x": -9553.46875,
+ "y": -914.75,
+ "z": 19812.28125
+ }
+ }
+ },
+ {
+ "title": "Tir - Eol Prou LW-L c8-127 (15Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Tir",
+ "coords": {
+ "x": -9532.9375,
+ "y": -923.4375,
+ "z": 19799.125
+ }
+ }
+ },
+ {
+ "title": "Eol Procul Centauri - Eol Prou LW-L c8-127 (12Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Eol Procul Centauri",
+ "coords": {
+ "x": -9521.875,
+ "y": -919.40625,
+ "z": 19802.09375
+ }
+ }
+ },
+ {
+ "title": "Aurora Astrum - Eol Prou LW-L c8-127 (28Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Aurora Astrum",
+ "coords": {
+ "x": -9533.25,
+ "y": -888.9375,
+ "z": 19796.90625
+ }
+ }
+ },
+ {
+ "title": "Centralis - Eol Prou LW-L c8-127 (22Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Centralis",
+ "coords": {
+ "x": -9516.15625,
+ "y": -924.5,
+ "z": 19795.875
+ }
+ }
+ },
+ {
+ "title": "Kojeara - Eol Prou LW-L c8-127 (17Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Kojeara",
+ "coords": {
+ "x": -9513.09375,
+ "y": -908.84375,
+ "z": 19814.28125
+ }
+ }
+ },
+ {
+ "title": "Clooku VJ-E b16-27 - Stuelou AT-J c25-24 (2105Ly)",
+ "prison": {
+ "name": "Stuelou AT-J c25-24",
+ "coords": {
+ "x": -6704.4375,
+ "y": -156.34375,
+ "z": 14101.875
+ }
+ },
+ "jump": {
+ "name": "Clooku VJ-E b16-27",
+ "coords": {
+ "x": -6151.59375,
+ "y": -409.625,
+ "z": 12086.78125
+ }
+ }
+ },
+ {
+ "title": "Gandharvi - Stuelou AT-J c25-24 (6Ly)",
+ "prison": {
+ "name": "Stuelou AT-J c25-24",
+ "coords": {
+ "x": -6704.4375,
+ "y": -156.34375,
+ "z": 14101.875
+ }
+ },
+ "jump": {
+ "name": "Gandharvi",
+ "coords": {
+ "x": -6703.5,
+ "y": -157.84375,
+ "z": 14108.03125
+ }
+ }
+ },
+ {
+ "title": "Signalis - Eol Prou LW-L c8-127 (28Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Signalis",
+ "coords": {
+ "x": -9509.75,
+ "y": -916.8125,
+ "z": 19788.53125
+ }
+ }
+ },
+ {
+ "title": "Luchtaine - Eol Prou LW-L c8-127 (18Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Luchtaine",
+ "coords": {
+ "x": -9523.3125,
+ "y": -914.46875,
+ "z": 19825.90625
+ }
+ }
+ },
+ {
+ "title": "Deriso - Eol Prou LW-L c8-127 (9Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Deriso",
+ "coords": {
+ "x": -9520.3125,
+ "y": -909.5,
+ "z": 19808.75
+ }
+ }
+ },
+ {
+ "title": "Helgoland - Eol Prou LW-L c8-127 (32Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Helgoland",
+ "coords": {
+ "x": -9510.21875,
+ "y": -908.5,
+ "z": 19783.78125
+ }
+ }
+ },
+ {
+ "title": "Eoch Flyuae QK-E d12-2118 - Eol Prou LW-L c8-127 (2081Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Eoch Flyuae QK-E d12-2118",
+ "coords": {
+ "x": -8633.78125,
+ "y": -700.8125,
+ "z": 17942.1875
+ }
+ }
+ },
+ {
+ "title": "Diggidiggi - Eol Prou LW-L c8-127 (26Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Diggidiggi",
+ "coords": {
+ "x": -9518.34375,
+ "y": -893.09375,
+ "z": 19822.90625
+ }
+ }
+ },
+ {
+ "title": "Macrath - Eol Prou LW-L c8-127 (19Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Macrath",
+ "coords": {
+ "x": -9545.15625,
+ "y": -909.9375,
+ "z": 19818.96875
+ }
+ }
+ },
+ {
+ "title": "Coeus - Eol Prou LW-L c8-127 (41Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Coeus",
+ "coords": {
+ "x": -9531.71875,
+ "y": -885.3125,
+ "z": 19839.21875
+ }
+ }
+ },
+ {
+ "title": "Eol Prou GE-A c1-291 - Eol Prou LW-L c8-127 (344Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Eol Prou GE-A c1-291",
+ "coords": {
+ "x": -9406.8125,
+ "y": -874.59375,
+ "z": 19490.09375
+ }
+ }
+ },
+ {
+ "title": "Ogmar - Eol Prou LW-L c8-127 (12Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Ogmar",
+ "coords": {
+ "x": -9534.0,
+ "y": -905.28125,
+ "z": 19802.03125
+ }
+ }
+ },
+ {
+ "title": "Kashyapa - Eol Prou LW-L c8-127 (2534Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Kashyapa",
+ "coords": {
+ "x": -8392.03125,
+ "y": -701.03125,
+ "z": 17555.125
+ }
+ }
+ },
+ {
+ "title": "Clooku AA-Q b37-41 - Stuelou AT-J c25-24 (1634Ly)",
+ "prison": {
+ "name": "Stuelou AT-J c25-24",
+ "coords": {
+ "x": -6704.4375,
+ "y": -156.34375,
+ "z": 14101.875
+ }
+ },
+ "jump": {
+ "name": "Clooku AA-Q b37-41",
+ "coords": {
+ "x": -6287.78125,
+ "y": -404.96875,
+ "z": 12541.40625
+ }
+ }
+ },
+ {
+ "title": "Stuelou VV-X c17-395 - Stuelou AT-J c25-24 (317Ly)",
+ "prison": {
+ "name": "Stuelou AT-J c25-24",
+ "coords": {
+ "x": -6704.4375,
+ "y": -156.34375,
+ "z": 14101.875
+ }
+ },
+ "jump": {
+ "name": "Stuelou VV-X c17-395",
+ "coords": {
+ "x": -6631.9375,
+ "y": -165.625,
+ "z": 13793.90625
+ }
+ }
+ },
+ {
+ "title": "Stuelou UT-E b17-51 - Stuelou AT-J c25-24 (721Ly)",
+ "prison": {
+ "name": "Stuelou AT-J c25-24",
+ "coords": {
+ "x": -6704.4375,
+ "y": -156.34375,
+ "z": 14101.875
+ }
+ },
+ "jump": {
+ "name": "Stuelou UT-E b17-51",
+ "coords": {
+ "x": -6569.46875,
+ "y": -198.34375,
+ "z": 13394.78125
+ }
+ }
+ },
+ {
+ "title": "Boelts ZN-Y b5-69 - Stuelou AT-J c25-24 (1742Ly)",
+ "prison": {
+ "name": "Stuelou AT-J c25-24",
+ "coords": {
+ "x": -6704.4375,
+ "y": -156.34375,
+ "z": 14101.875
+ }
+ },
+ "jump": {
+ "name": "Boelts ZN-Y b5-69",
+ "coords": {
+ "x": -7360.71875,
+ "y": -335.0,
+ "z": 15705.0625
+ }
+ }
+ },
+ {
+ "title": "PMD2009 48 - California Sector CQ-Y c5 (971Ly)",
+ "prison": {
+ "name": "California Sector CQ-Y c5",
+ "coords": {
+ "x": -350.375,
+ "y": -245.53125,
+ "z": -950.625
+ }
+ },
+ "jump": {
+ "name": "PMD2009 48",
+ "coords": {
+ "x": 594.90625,
+ "y": -431.4375,
+ "z": -1071.78125
+ }
+ }
+ },
+ {
+ "title": "Gria Drye IR-F a38-10 - Omega Sector OD-S b4-0 (462Ly)",
+ "prison": {
+ "name": "Omega Sector OD-S b4-0",
+ "coords": {
+ "x": -1456.8125,
+ "y": -84.8125,
+ "z": 5306.9375
+ }
+ },
+ "jump": {
+ "name": "Gria Drye IR-F a38-10",
+ "coords": {
+ "x": -1604.9375,
+ "y": -26.09375,
+ "z": 5740.71875
+ }
+ }
+ },
+ {
+ "title": "Clooku QA-E c28-713 - Stuelou AT-J c25-24 (1195Ly)",
+ "prison": {
+ "name": "Stuelou AT-J c25-24",
+ "coords": {
+ "x": -6704.4375,
+ "y": -156.34375,
+ "z": 14101.875
+ }
+ },
+ "jump": {
+ "name": "Clooku QA-E c28-713",
+ "coords": {
+ "x": -6425.75,
+ "y": -256.46875,
+ "z": 12944.59375
+ }
+ }
+ },
+ {
+ "title": "Boelts UB-P b24-98 - Stuelou AT-J c25-24 (2182Ly)",
+ "prison": {
+ "name": "Stuelou AT-J c25-24",
+ "coords": {
+ "x": -6704.4375,
+ "y": -156.34375,
+ "z": 14101.875
+ }
+ },
+ "jump": {
+ "name": "Boelts UB-P b24-98",
+ "coords": {
+ "x": -7542.1875,
+ "y": -359.15625,
+ "z": 16106.125
+ }
+ }
+ },
+ {
+ "title": "Dryooe Flyou NQ-G b27-103 - Eol Prou LW-L c8-127 (1194Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Dryooe Flyou NQ-G b27-103",
+ "coords": {
+ "x": -9082.5,
+ "y": -697.25,
+ "z": 18723.4375
+ }
+ }
+ },
+ {
+ "title": "Dryio Flyuae KV-P b8-112 - Eol Prou LW-L c8-127 (1638Ly)",
+ "prison": {
+ "name": "Eol Prou LW-L c8-127",
+ "coords": {
+ "x": -9528.84375,
+ "y": -913.25,
+ "z": 19809.3125
+ }
+ },
+ "jump": {
+ "name": "Dryio Flyuae KV-P b8-112",
+ "coords": {
+ "x": -8855.0,
+ "y": -698.53125,
+ "z": 18331.71875
+ }
+ }
+ },
+ {
+ "title": "Stuemeae FG-Y d7561 - Stuemeae BA-A d955 (6Ly)",
+ "prison": {
+ "name": "Stuemeae BA-A d955",
+ "coords": {
+ "x": 27.84375,
+ "y": -22.4375,
+ "z": 25894.59375
+ }
+ },
+ "jump": {
+ "name": "Stuemeae FG-Y d7561",
+ "coords": {
+ "x": 28.6875,
+ "y": -19.78125,
+ "z": 25899.6875
+ }
+ }
+ },
+ {
+ "title": "Blua Eaec WW-E c14-1293 - Stuelou AT-J c25-24 (844Ly)",
+ "prison": {
+ "name": "Stuelou AT-J c25-24",
+ "coords": {
+ "x": -6704.4375,
+ "y": -156.34375,
+ "z": 14101.875
+ }
+ },
+ "jump": {
+ "name": "Blua Eaec WW-E c14-1293",
+ "coords": {
+ "x": -6989.375,
+ "y": -180.71875,
+ "z": 14895.8125
+ }
+ }
+ }
+]
\ No newline at end of file
diff --git a/dcoh.html b/dcoh.html
new file mode 100644
index 0000000..8cd0d8a
--- /dev/null
+++ b/dcoh.html
@@ -0,0 +1,73 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ CanonnED3D - Maps
+
+
+
+
+
+
+
+
+
+
+
+

+
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/dcoh_headless.html b/dcoh_headless.html
new file mode 100644
index 0000000..92a2476
--- /dev/null
+++ b/dcoh_headless.html
@@ -0,0 +1,68 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ CanonnED3D - Maps
+
+
+
+
+
+
+
+
+

+
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/faction_data.html b/faction_data.html
new file mode 100644
index 0000000..2754ede
--- /dev/null
+++ b/faction_data.html
@@ -0,0 +1,73 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ CanonnED3D - Maps
+
+
+
+
+
+
+
+
+
+
+
+

+
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/faction_res_data.html b/faction_res_data.html
new file mode 100644
index 0000000..fcc25ac
--- /dev/null
+++ b/faction_res_data.html
@@ -0,0 +1,73 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ CanonnED3D - Maps
+
+
+
+
+
+
+
+
+
+
+
+

+
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/fg-data.html b/fg-data.html
new file mode 100644
index 0000000..edf1a74
--- /dev/null
+++ b/fg-data.html
@@ -0,0 +1,71 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ CanonnED3D - Maps
+
+
+
+
+
+
+
+
+
+
+
+

+
+
+
+
+
+
+
+
+
+
diff --git a/fm-data.html b/fm-data.html
new file mode 100644
index 0000000..e814ad6
--- /dev/null
+++ b/fm-data.html
@@ -0,0 +1,71 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ CanonnED3D - Maps
+
+
+
+
+
+
+
+
+
+
+
+

+
+
+
+
+
+
+
+
+
+
diff --git a/gb-data.html b/gb-data.html
new file mode 100644
index 0000000..a9d2200
--- /dev/null
+++ b/gb-data.html
@@ -0,0 +1,71 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ CanonnED3D - Maps
+
+
+
+
+
+
+
+
+
+
+
+

+
+
+
+
+
+
+
+
+
+
diff --git a/gen-data.html b/gen-data.html
new file mode 100644
index 0000000..eeb3f5a
--- /dev/null
+++ b/gen-data.html
@@ -0,0 +1,71 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ CanonnED3D - Maps
+
+
+
+
+
+
+
+
+
+
+
+

+
+
+
+
+
+
+
+
+
+
diff --git a/geology-combo.html b/geology-combo.html
new file mode 100644
index 0000000..da7c67f
--- /dev/null
+++ b/geology-combo.html
@@ -0,0 +1,71 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ CanonnED3D - Maps
+
+
+
+
+
+
+
+
+
+
+
+

+
+
+
+
+
+
+
+
+
+
diff --git a/gnosis_data.html b/gnosis_data.html
new file mode 100644
index 0000000..7f6c687
--- /dev/null
+++ b/gnosis_data.html
@@ -0,0 +1,74 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ CanonnED3D - Maps
+
+
+
+
+
+
+
+
+
+
+
+

+
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/gr-data.html b/gr-data.html
new file mode 100644
index 0000000..82b0756
--- /dev/null
+++ b/gr-data.html
@@ -0,0 +1,71 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ CanonnED3D - Maps
+
+
+
+
+
+
+
+
+
+
+
+

+
+
+
+
+
+
+
+
+
+
diff --git a/guardians-combo.html b/guardians-combo.html
new file mode 100644
index 0000000..c22e002
--- /dev/null
+++ b/guardians-combo.html
@@ -0,0 +1,71 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ CanonnED3D - Maps
+
+
+
+
+
+
+
+
+
+
+
+

+
+
+
+
+
+
+
+
+
+
diff --git a/gv-data.html b/gv-data.html
new file mode 100644
index 0000000..acc64d6
--- /dev/null
+++ b/gv-data.html
@@ -0,0 +1,71 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ CanonnED3D - Maps
+
+
+
+
+
+
+
+
+
+
+
+

+
+
+
+
+
+
+
+
+
+
diff --git a/gy-data.html b/gy-data.html
new file mode 100644
index 0000000..8c6839e
--- /dev/null
+++ b/gy-data.html
@@ -0,0 +1,71 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ CanonnED3D - Maps
+
+
+
+
+
+
+
+
+
+
+
+

+
+
+
+
+
+
+
+
+
+
diff --git a/hyperdiction_data.html b/hyperdiction_data.html
new file mode 100644
index 0000000..a80d3bf
--- /dev/null
+++ b/hyperdiction_data.html
@@ -0,0 +1,73 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ CanonnED3D - Maps
+
+
+
+
+
+
+
+
+
+
+
+

+
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/img/Canonn-Logo.gif b/img/Canonn-Logo.gif
new file mode 100644
index 0000000..e530ff1
Binary files /dev/null and b/img/Canonn-Logo.gif differ
diff --git a/img/bear_thumbnail.png b/img/bear_thumbnail.png
new file mode 100644
index 0000000..4987245
Binary files /dev/null and b/img/bear_thumbnail.png differ
diff --git a/img/bowl_thumbnail.png b/img/bowl_thumbnail.png
new file mode 100644
index 0000000..4b1ffae
Binary files /dev/null and b/img/bowl_thumbnail.png differ
diff --git a/img/crossroads_thumbnail.png b/img/crossroads_thumbnail.png
new file mode 100644
index 0000000..317380d
Binary files /dev/null and b/img/crossroads_thumbnail.png differ
diff --git a/img/favicon.ico b/img/favicon.ico
new file mode 100644
index 0000000..3b1ce9f
Binary files /dev/null and b/img/favicon.ico differ
diff --git a/img/fistbump_thumbnail.png b/img/fistbump_thumbnail.png
new file mode 100644
index 0000000..808c4b1
Binary files /dev/null and b/img/fistbump_thumbnail.png differ
diff --git a/img/hammerbot_thumbnail.png b/img/hammerbot_thumbnail.png
new file mode 100644
index 0000000..8f9e9a9
Binary files /dev/null and b/img/hammerbot_thumbnail.png differ
diff --git a/img/lacrosse_thumbnail_001.png b/img/lacrosse_thumbnail_001.png
new file mode 100644
index 0000000..967c677
Binary files /dev/null and b/img/lacrosse_thumbnail_001.png differ
diff --git a/img/soontill.png b/img/soontill.png
new file mode 100644
index 0000000..80081ca
Binary files /dev/null and b/img/soontill.png differ
diff --git a/img/turtle_thumbnail.png b/img/turtle_thumbnail.png
new file mode 100644
index 0000000..4742c81
Binary files /dev/null and b/img/turtle_thumbnail.png differ
diff --git a/include/nav.html b/include/nav.html
new file mode 100644
index 0000000..2f38d5f
--- /dev/null
+++ b/include/nav.html
@@ -0,0 +1,217 @@
+
+
diff --git a/index.html b/index.html
new file mode 100644
index 0000000..3b2e841
--- /dev/null
+++ b/index.html
@@ -0,0 +1,71 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ CanonnED3D - Maps
+
+
+
+
+
+
+
+
+
+
+
+

+
+
+
+
+
+
+
+
+
+
diff --git a/js/components/action.class.js b/js/components/action.class.js
new file mode 100644
index 0000000..1095c9b
--- /dev/null
+++ b/js/components/action.class.js
@@ -0,0 +1,627 @@
+
+var Action = {
+
+ 'cursorSel' : null,
+ 'cursorHover' : null,
+ 'cursorScale' : 1,
+
+
+ 'cursor' : {
+ 'selection' : null,
+ 'hover' : null,
+ },
+
+ 'mouseVector' : null,
+ 'raycaster' : null,
+ 'oldSel' : null,
+ 'objHover' : null,
+ 'mouseUpDownTimer' : null,
+ 'mouseHoverTimer' : null,
+ 'animPosition' : null,
+
+ 'prevScale' : null,
+
+ 'pointCastRadius' : 2,
+
+ 'pointsHighlight' : [],
+
+ /**
+ * Init Raycaster for events on Systems
+ */
+
+ 'init' : function() {
+
+ this.mouseVector = new THREE.Vector3();
+ this.raycaster = new THREE.Raycaster();
+
+ var obj = this;
+
+ container.addEventListener('mousedown', function(e){obj.onMouseDown(e,obj);}, false);
+ container.addEventListener('mouseup', function(e){obj.onMouseUp(e,obj);}, false);
+ container.addEventListener('mousemove', function(e){obj.onMouseHover(e,obj);}, false);
+
+ container.addEventListener('mousewheel', this.stopWinScroll, false );
+ container.addEventListener('DOMMouseScroll', this.stopWinScroll, false ); // FF
+
+
+ if(Ed3d.showNameNear) {
+ console.log('Launch EXPERIMENTAL func');
+ window.setInterval(function(){
+ obj.highlightAroundCamera(obj);
+ }, 1000);
+ }
+ },
+
+ /**
+ * Stop window scroll when mouse on scene
+ */
+
+ 'stopWinScroll' : function (event) {
+ event.preventDefault();
+ event.stopPropagation();
+ },
+
+ /**
+ * Update point click radius: increase radius with distance
+ */
+
+ 'updatePointClickRadius' : function (radius) {
+ radius = Math.round(radius);
+ if(radius<2) radius = 2;
+ if(this.pointCastRadius == radius) return;
+ this.pointCastRadius = radius;
+ },
+
+ /**
+ * Update particle size on zoom in/out
+ */
+
+ 'sizeOnScroll' : function (scale) {
+
+ if(System.particle == undefined || scale<=0) return;
+
+ var minScale = Ed3d.effectScaleSystem[0];
+ var maxScale = Ed3d.effectScaleSystem[1];
+ var newScale = scale*20;
+
+ if(this.prevScale == newScale) return;
+ this.prevScale = newScale;
+
+
+ if(newScale>maxScale) newScale = maxScale;
+ if(newScale 0) {
+
+ //-- Highlight new selection
+
+ for( var i = 0; i < intersects.length; i++ ) {
+
+ if(count>limit) return;
+
+ var intersection = intersects[ i ];
+ if(intersection.object.clickable) {
+
+ var indexPoint = intersection.index;
+ var selPoint = intersection.object.geometry.vertices[indexPoint];
+
+ if(selPoint.visible) {
+ var textAdd = selPoint.name;
+ var textId = 'highlight_'+indexPoint
+ if(obj.pointsHighlight.indexOf(textId) == -1) {
+
+ HUD.addText(textId, textAdd, 0, 4, 0, 1, selPoint, true);
+
+ obj.pointsHighlight.push(textId);
+ }
+ newSel[textId] = textId;
+ }
+
+ count++;
+
+ }
+ }
+
+ }
+
+ //-- Remove old selection
+
+ $.each(obj.pointsHighlight, function(key, item) {
+ if(newSel[item] == undefined) {
+ var object = Ed3d.textSel[item];
+ if(object != undefined) {
+ scene.remove(object);
+ Ed3d.textSel.splice(item, 1);
+ obj.pointsHighlight.splice(key, 1);
+ }
+ }
+ });
+
+ },
+
+
+ /**
+ * Mouse Hover
+ */
+
+ 'onMouseHover' : function (e, obj) {
+
+ e.preventDefault();
+
+ var position = $('#ed3dmap').offset();
+ var scrollPos = $(window).scrollTop();
+ position.top -= scrollPos;
+
+ obj.mouseVector = new THREE.Vector3(
+ ( ( e.clientX - position.left ) / renderer.domElement.width ) * 2 - 1,
+ - ( ( e.clientY - position.top ) / renderer.domElement.height ) * 2 + 1,
+ 1);
+
+ obj.mouseVector.unproject(camera);
+ obj.raycaster = new THREE.Raycaster(camera.position, obj.mouseVector.sub(camera.position).normalize());
+ obj.raycaster.params.Points.threshold = obj.pointCastRadius;
+
+ // create an array containing all objects in the scene with which the ray intersects
+ var intersects = obj.raycaster.intersectObjects(scene.children);
+ if (intersects.length > 0) {
+
+ for( var i = 0; i < intersects.length; i++ ) {
+ var intersection = intersects[ i ];
+ if(intersection.object.clickable) {
+
+ var indexPoint = intersection.index;
+ var selPoint = intersection.object.geometry.vertices[indexPoint];
+
+ if(selPoint.visible) {
+ Action.hoverOnObj(indexPoint);
+ return;
+ }
+ }
+ }
+
+
+
+ } else {
+ Action.outOnObj();
+ }
+
+ },
+
+ 'hoverOnObj' : function (indexPoint) {
+
+ if(this.objHover == indexPoint) return;
+ this.outOnObj();
+
+ this.objHover = indexPoint;
+
+ var sel = System.particleGeo.vertices[indexPoint];
+ this.addCursorOnHover(sel);
+
+ },
+
+ 'outOnObj' : function () {
+
+ if(this.objHover === null || System.particleGeo.vertices[this.objHover] == undefined)
+ return;
+
+ this.objHover = null;
+ this.cursor.hover.visible = false;
+
+ },
+
+ /**
+ * On system click
+ */
+
+ 'onMouseDown' : function (e, obj) {
+
+ obj.mouseUpDownTimer = Date.now();
+
+ },
+
+
+ /**
+ * On system click
+ */
+
+ 'onMouseUp' : function (e, obj) {
+
+ e.preventDefault();
+
+ //-- If long clic down, don't do anything
+
+ var difference = (Date.now()-obj.mouseUpDownTimer)/1000;
+ if (difference > 0.2) {
+ obj.mouseUpDownTimer = null;
+ return;
+ }
+ obj.mouseUpDownTimer = null;
+
+ //-- Raycast object
+
+ var position = $('#ed3dmap').offset();
+ var scrollPos = $(window).scrollTop();
+ position.top -= scrollPos;
+
+ obj.mouseVector = new THREE.Vector3(
+ ( ( e.clientX - position.left ) / renderer.domElement.width ) * 2 - 1,
+ - ( ( e.clientY - position.top ) / renderer.domElement.height ) * 2 + 1,
+ 1);
+
+
+ obj.mouseVector.unproject(camera);
+ obj.raycaster = new THREE.Raycaster(camera.position, obj.mouseVector.sub(camera.position).normalize());
+ obj.raycaster.params.Points.threshold = obj.pointCastRadius;
+
+
+ // create an array containing all objects in the scene with which the ray intersects
+ var intersects = obj.raycaster.intersectObjects(scene.children);
+ if (intersects.length > 0) {
+
+ for( var i = 0; i < intersects.length; i++ ) {
+ var intersection = intersects[ i ];
+ if(intersection.object.clickable) {
+
+ var indexPoint = intersection.index;
+ var selPoint = intersection.object.geometry.vertices[indexPoint];
+
+ if(selPoint.visible) {
+ $('#hud #infos').html(
+ ""+selPoint.name+"
"
+ );
+
+ var isMove = obj.moveToObj(indexPoint, selPoint);
+
+ var opt = [ selPoint.name ];
+
+ var optInfos = (selPoint.infos != undefined) ? selPoint.infos : null;
+ var optUrl = (selPoint.url != undefined) ? selPoint.url : null;
+
+ $(document).trigger( "systemClick", [ selPoint.name, optInfos, optUrl ] );
+
+ if(isMove) return;
+ }
+
+ }
+
+ if(intersection.object.showCoord) {
+
+ $('#debug').html(Math.round(intersection.point.x)+' , '+Math.round(-intersection.point.z));
+
+ //Route.addPointToRoute(Math.round(intersection.point.x),0,Math.round(-intersection.point.z));
+
+ }
+ }
+
+ }
+
+ obj.disableSelection();
+
+ },
+
+ /**
+ * Move to the next visible system
+ *
+ * @param {int} indexPoint
+ */
+
+ 'moveNextPrev' : function (indexPoint, increment) {
+
+ var find = false;
+ while(!find) {
+
+ //-- If next|previous is undefined, loop to the first|last
+ if (indexPoint < 0) indexPoint = System.particleGeo.vertices.length-1;
+ else if (System.particleGeo.vertices[indexPoint] == undefined) indexPoint = 0;
+
+ if(System.particleGeo.vertices[indexPoint].visible == true) {
+ find = true;
+ } else {
+ indexPoint += increment
+ }
+ }
+
+
+ //-- Move to
+ var selPoint = System.particleGeo.vertices[indexPoint];
+ this.moveToObj(indexPoint, selPoint);
+
+ },
+
+ /**
+ * Disable current selection
+ */
+
+ 'disableSelection' : function () {
+
+ if(this.cursor.selection == null) return;
+
+ this.oldSel = null;
+ this.cursor.selection.visible = false;
+
+ $('#hud #infos').html('');
+
+ },
+
+ /**
+ * Move to inital position without animation
+ */
+ 'moveInitalPositionNoAnim' : function (timer) {
+
+ var cam = [Ed3d.playerPos[0], Ed3d.playerPos[1]+500, -Ed3d.playerPos[2]+500];
+ if(Ed3d.cameraPos != null) {
+ cam = [Ed3d.cameraPos[0], Ed3d.cameraPos[1], -Ed3d.cameraPos[2]];
+ }
+
+ var moveTo = {
+ x: cam[0], y: cam[1], z: cam[2],
+ mx: Ed3d.playerPos[0], my: Ed3d.playerPos[1] , mz: -Ed3d.playerPos[2]
+ };
+ camera.position.set(moveTo.x, moveTo.y, moveTo.z);
+ controls.target.set(moveTo.mx, moveTo.my, moveTo.mz);
+
+ },
+
+ /**
+ * Move to inital position
+ */
+ 'moveInitalPosition' : function (timer) {
+
+ if(timer == undefined) timer = 800;
+
+ this.disableSelection();
+
+ //-- Move camera to initial position
+
+ var moveFrom = {
+ x: camera.position.x, y: camera.position.y , z: camera.position.z,
+ mx: controls.target.x, my: controls.target.y , mz: controls.target.z
+ };
+
+ //-- Move to player position if defined, else move to Sol
+ var cam = [Ed3d.playerPos[0], Ed3d.playerPos[1]+500, -Ed3d.playerPos[2]+500]
+ if(Ed3d.cameraPos != null) {
+ cam = [Ed3d.cameraPos[0], Ed3d.cameraPos[1], -Ed3d.cameraPos[2]];
+ }
+
+ var moveCoords = {
+ x: cam[0], y: cam[1], z: cam[2],
+ mx: Ed3d.playerPos[0], my: Ed3d.playerPos[1] , mz: -Ed3d.playerPos[2]
+ };
+
+ controls.enabled = false;
+
+ //-- Remove previous anim
+ if(Ed3d.tween != null) {
+ TWEEN.removeAll();
+ }
+
+ //-- Launch anim
+ Ed3d.tween = new TWEEN.Tween(moveFrom, {override:true}).to(moveCoords, timer)
+ .start()
+ .onUpdate(function () {
+ camera.position.set(moveFrom.x, moveFrom.y, moveFrom.z);
+ controls.target.set(moveFrom.mx, moveFrom.my, moveFrom.mz);
+ })
+ .onComplete(function () {
+ controls.enabled = true;
+ controls.update();
+ });
+
+ },
+
+
+ /**
+ * Move camera to a system
+ *
+ * @param {int} index - The system index
+ * @param {object} obj - System datas
+ */
+
+ 'moveToObj' : function (index, obj) {
+
+ if (this.oldSel !== null && this.oldSel == index) return false;
+
+ controls.enabled = false;
+
+ HUD.setInfoPanel(index, obj);
+
+ if(obj.infos != undefined) HUD.openHudDetails();
+
+
+ this.oldSel = index;
+ var goX = obj.x;
+ var goY = obj.y;
+ var goZ = obj.z;
+
+ //-- If in far view reset to classic view
+ disableFarView(25, false);
+
+ //-- Move grid to object
+ this.moveGridTo(goX, goY, goZ);
+
+ //-- Move camera to target (Smooth move using Tween)
+
+ var moveFrom = {
+ x: camera.position.x, y: camera.position.y , z: camera.position.z,
+ mx: controls.target.x, my: controls.target.y , mz: controls.target.z
+ };
+ var moveCoords = {
+ x: goX, y: goY + 15, z: goZ + 15,
+ mx: goX, my: goY , mz: goZ
+ };
+
+ Ed3d.tween = new TWEEN.Tween(moveFrom, {override:true}).to(moveCoords, 800)
+ .start()
+ .onUpdate(function () {
+ camera.position.set(moveFrom.x, moveFrom.y, moveFrom.z);
+ controls.target.set(moveFrom.mx, moveFrom.my, moveFrom.mz);
+ })
+ .onComplete(function () {
+ controls.update();
+ });
+
+ //-- 3D Cursor on selected object
+
+ obj.material = Ed3d.material.selected;
+
+ this.addCursorOnSelect(goX, goY, goZ);
+
+ //-- Add text
+ var textAdd = obj.name;
+ var textAddC = Math.round(goX) + ', ' + Math.round(goY) + ', ' + Math.round(-goZ);
+
+ HUD.addText('system', textAdd, 8, 20, 0, 6, this.cursor.selection);
+ HUD.addText('coords', textAddC, 8, 15, 0, 3, this.cursor.selection);
+
+ controls.enabled = true;
+
+ return true;
+
+ },
+
+ /**
+ * Create a cursor on selected system
+ *
+ * @param {number} x
+ * @param {number} y
+ * @param {number} z
+ */
+
+ 'addCursorOnSelect' : function (x, y, z) {
+
+ if(this.cursor.selection == null) {
+ this.cursor.selection = new THREE.Object3D();
+
+ //-- Ring around the system
+ var geometryL = new THREE.TorusGeometry( 8, 0.4, 3, 20 );
+
+ var selection = new THREE.Mesh(geometryL, Ed3d.material.selected);
+ //selection.position.set(x, y, z);
+ selection.rotation.x = Math.PI / 2;
+
+ this.cursor.selection.add(selection);
+
+ //-- Create a cone on the selection
+ var geometryCone = new THREE.CylinderGeometry(0, 5, 16, 4, 1, false);
+ var cone = new THREE.Mesh(geometryCone, Ed3d.material.selected);
+ cone.position.set(0, 20, 0);
+ cone.rotation.x = Math.PI;
+ this.cursor.selection.add(cone);
+
+ //-- Inner cone
+ var geometryConeInner = new THREE.CylinderGeometry(0, 3.6, 16, 4, 1, false);
+ var coneInner = new THREE.Mesh(geometryConeInner, Ed3d.material.black);
+ coneInner.position.set(0, 20.2, 0);
+ coneInner.rotation.x = Math.PI;
+ this.cursor.selection.add(coneInner);
+
+
+
+ scene.add(this.cursor.selection);
+ }
+
+ this.cursor.selection.visible = true;
+ this.cursor.selection.position.set(x, y, z);
+ this.cursor.hover.scale.set(this.cursorScale, this.cursorScale, this.cursorScale);
+
+ },
+
+ /**
+ * Create a cursor on hover
+ *
+ * @param {number} x
+ * @param {number} y
+ * @param {number} z
+ */
+
+ 'addCursorOnHover' : function (obj) {
+
+ if(this.cursor.hover == null) {
+ this.cursor.hover = new THREE.Object3D();
+
+ //-- Ring around the system
+ var geometryL = new THREE.TorusGeometry( 6, 0.4, 3, 20 );
+
+ var selection = new THREE.Mesh(geometryL, Ed3d.material.grey);
+ selection.rotation.x = Math.PI / 2;
+
+ this.cursor.hover.add(selection);
+
+ scene.add(this.cursor.hover);
+ }
+
+ this.cursor.hover.position.set(obj.x, obj.y, obj.z);
+ this.cursor.hover.visible = true;
+ this.cursor.hover.scale.set(this.cursorScale, this.cursorScale, this.cursorScale);
+
+ //-- Add text
+
+ var textAdd = obj.name;
+ HUD.addText('system_hover', textAdd, 0, 4, 0, 3, this.cursor.hover);
+
+ },
+
+ /**
+ * Update cursor size with camera distance
+ *
+ * @param {number} distance
+ */
+
+ 'updateCursorSize' : function (scale) {
+
+ var obj = this;
+
+ $.each(this.cursor, function(key, cur) {
+ if(cur != null) {
+ cur.scale.set(scale, scale, scale);
+ }
+ });
+
+ this.cursorScale = scale
+
+ },
+
+ /**
+ * Move grid to selection
+ *
+ * @param {number} goX
+ * @param {number} goY
+ * @param {number} goZ
+ */
+
+ 'moveGridTo' : function (goX, goY, goZ) {
+ var posX = Math.floor(goX/1000)*1000;
+ var posY = Math.floor(goY);
+ var posZ = Math.floor(goZ/1000)*1000;
+
+ if(!Ed3d.grid1H.fixed) Ed3d.grid1H.obj.position.set(posX, posY, posZ);
+ if(!Ed3d.grid1K.fixed) Ed3d.grid1K.obj.position.set(posX, posY, posZ);
+ if(!Ed3d.grid1XL.fixed) Ed3d.grid1XL.obj.position.set(posX, posY, posZ);
+
+ }
+
+
+
+}
\ No newline at end of file
diff --git a/js/components/galaxy.class.js b/js/components/galaxy.class.js
new file mode 100644
index 0000000..be05b4a
--- /dev/null
+++ b/js/components/galaxy.class.js
@@ -0,0 +1,389 @@
+
+var Galaxy = {
+
+
+ 'obj' : null,
+ 'infos' : null,
+ 'milkyway' : [],
+ 'milkyway2D' : null,
+ 'backActive' : true,
+ 'colors' : [],
+
+ 'x' : 25,
+ 'y' : -21,
+ 'z' : 25900,
+
+ //-- Objects
+ 'Action' : null,
+
+ /**
+ * Remove galaxy
+ */
+
+ 'remove' : function() {
+
+ //scene.remove(this.milkyway[0]);
+ //scene.remove(this.milkyway[1]);
+ scene.remove(this.milkyway2D);
+
+ },
+
+ 'addGalaxyCenter' : function () {
+
+ var objVal = new Object;
+ objVal.name = 'Sagittarius A*';
+ objVal.coords = {'x':this.y,'y':this.y,'z':this.z};
+ objVal.cat = [];
+
+ this.obj = System.create(objVal, true);
+
+
+ var sprite = new THREE.Sprite( Ed3d.material.glow_2 );
+ sprite.scale.set(50, 40, 2.0);
+ this.obj.add(sprite); /// this centers the glow at the mesh
+
+ this.createParticles();
+ this.add2DPlane();
+
+ },
+
+ 'createParticles' : function () {
+
+ var img = new Image();
+ var obj = this;
+
+ img.onload = function () {
+
+ //get height data from img
+ obj.getHeightData(img, obj);
+
+
+ //-- If using start animation: launch it
+ if(Ed3d.startAnim) {
+ camera.position.set(-10000, 40000, 50000);
+ Action.moveInitalPosition(4000);
+ } else {
+ Action.moveInitalPositionNoAnim();
+ }
+
+ };
+
+ //-- load img source
+ img.src = Ed3d.basePath + "textures/heightmap7.jpg";
+
+ //-- Add optional infos
+ this.showGalaxyInfos();
+
+ },
+
+ /**
+ * Add 2D image plane
+ */
+
+ 'showGalaxyInfos' : function() {
+
+ if(!Ed3d.showGalaxyInfos) return;
+
+ this.infos = new THREE.Object3D();
+ var obj = this;
+
+ $.getJSON(Ed3d.basePath + "data/milkyway-ed.json", function(data) {
+
+ $.each(data.quadrants, function(key, val) {
+
+ obj.addText(key,val.x,-100,val.z,val.rotate);
+
+ });
+
+ $.each(data.arms, function(key, val) {
+
+ $.each(val, function(keyCh, valCh) {
+ obj.addText(key,valCh.x,0,valCh.z,valCh.rotate,300,true);
+ });
+
+ });
+
+ $.each(data.gaps, function(key, val) {
+
+ $.each(val, function(keyCh, valCh) {
+ obj.addText(key,valCh.x,0,valCh.z,valCh.rotate,160,true);
+ });
+
+ });
+
+ $.each(data.others, function(key, val) {
+
+ $.each(val, function(keyCh, valCh) {
+ obj.addText(key,valCh.x,0,valCh.z,valCh.rotate,160,true);
+ });
+
+ });
+
+
+ }).done(function() {
+
+ scene.add(obj.infos);
+
+ });
+
+ },
+
+ /**
+ * Show additional galaxy infos
+ */
+ 'infosShow' : function() {
+ if(this.infos == null) this.showGalaxyInfos();
+ if(this.infos !== null) this.infos.visible = Ed3d.showGalaxyInfos;
+ },
+
+ /**
+ * Show additional galaxy infos
+ */
+ 'infosHide' : function() {
+ if(this.infos !== null) this.infos.visible = false;
+ },
+
+ /**
+ * Appli opacity for Milky Way info based on distance
+ */
+
+ 'infosUpdateCallback' : function(scale) {
+
+ if(!Ed3d.showGalaxyInfos || this.infos == null) return;
+
+ scale -= 70;
+
+ var opacity = Math.round(scale/10)/10;
+ if(opacity<0) opacity = 0;
+ if(opacity>0.8) opacity = 0.8;
+ if(this.infos.previousOpacity == opacity) return;
+
+ var opacityMiddle = 1.1-opacity;
+ if(opacityMiddle<=0.4) opacityMiddle = 0.2;
+
+ for( var i = 0; i < this.infos.children.length; i++ ) {
+ var txt = this.infos.children[ i ];
+ txt.material.opacity = (!txt.revert) ? opacity : opacityMiddle;
+ }
+
+ this.infos.previousOpacity = opacity;
+
+ },
+
+ /**
+ * Add 2D image plane
+ */
+
+ 'add2DPlane' : function() {
+
+ var texloader = new THREE.TextureLoader();
+
+ //-- Load textures
+ var back2D = texloader.load(Ed3d.basePath + "textures/heightmap7.jpg");
+
+
+ var floorMaterial = new THREE.MeshBasicMaterial( {
+ map: back2D,
+ transparent: true,
+ opacity: 0.4,
+ blending: THREE.AdditiveBlending,
+ depthWrite: false,
+ side: THREE.DoubleSide
+ } );
+
+ var floorGeometry = new THREE.PlaneGeometry(104000, 104000, 1, 1);
+ this.milkyway2D = new THREE.Mesh(floorGeometry, floorMaterial);
+ this.milkyway2D.position.set(this.x, this.y, -this.z);
+ this.milkyway2D.rotation.x = -Math.PI / 2;
+ this.milkyway2D.showCoord = true;
+
+ scene.add(this.milkyway2D);
+
+ },
+
+ /**
+ * Add Shape text
+ */
+
+ 'addText' : function(textShow, x, y, z, rot, size, revert) {
+
+ if(revert==undefined) revert = false;
+ if(size==undefined) size = 450;
+ textShow = textShow.toUpperCase();
+
+ var textShapes = THREE.FontUtils.generateShapes(textShow, {
+ 'font': 'helvetiker',
+ 'weight': 'normal',
+ 'style': 'normal',
+ 'size': size,
+ 'curveSegments': 12
+ });
+
+ var textGeo = new THREE.ShapeGeometry(textShapes);
+
+ var textMesh = new THREE.Mesh(textGeo, new THREE.MeshBasicMaterial({
+ color: 0x999999,
+ transparent: true,
+ opacity: 0.7,
+ blending: THREE.AdditiveBlending,
+ depthWrite: false
+ }));
+
+ textMesh.geometry = textGeo;
+ textMesh.geometry.needsUpdate = true;
+
+ //x -= Math.round(textShow.length*400/2);
+ var middleTxt = Math.round(size/2);
+ z -= middleTxt;
+
+ textMesh.rotation.x = -Math.PI / 2;
+ textMesh.geometry.applyMatrix( new THREE.Matrix4().makeTranslation(-Math.round(textShow.length*size/2), 0, -middleTxt) );
+ if(rot != 0) {
+ textMesh.rotateOnAxis (new THREE.Vector3( 0, 0, 1 ), Math.PI * (rot) / 180);
+ }
+ textMesh.position.set(x, y, -z);
+
+ textMesh.revert = revert;
+
+ this.infos.add(textMesh);
+
+ },
+
+ /**
+ * Create a particle cloud milkyway from an image
+ *
+ * @param {Image} img - Image object
+ */
+
+ 'getHeightData' : function(img, obj) {
+
+ var particles = new THREE.Geometry;
+ var particlesBig = new THREE.Geometry;
+
+ //-- Get pixels from milkyway image
+
+ var canvas = document.createElement( 'canvas' );
+ canvas.width = img.width;
+ canvas.height = img.height;
+ var context = canvas.getContext( '2d' );
+
+ var size = img.width * img.height;
+
+ context.drawImage(img,0,0);
+
+ var imgd = context.getImageData(0, 0, img.width, img.height);
+ var pix = imgd.data;
+
+ //-- Build galaxy from image data
+
+ var j=0;
+ var min = 8;
+ var nb = 0;
+ var maxDensity = 15;
+
+ //var scaleImg = 16.4;
+ var scaleImg = 21;
+
+ var colorsBig = [];
+ var nbBig = 0;
+
+ for (var i = 0; i 0.5) i += 8;
+
+
+ var all = pix[i]+pix[i+1]+pix[i+2];
+
+ var avg = Math.round((pix[i]+pix[i+1]+pix[i+2])/3);
+
+ if(avg>min) {
+
+ var x = scaleImg*((i / 4) % img.width);
+ var z = scaleImg*(Math.floor((i / 4) / img.height));
+
+ var density = Math.floor((pix[i]-min)/10);
+ if(density>maxDensity) density = maxDensity;
+
+ var add = Math.ceil(density/maxDensity*2);
+ for (var y = -density; y < density; y = y+add) {
+
+ var particle = new THREE.Vector3(
+ x+((Math.random()-0.5) * 25),
+ (y*10)+((Math.random()-0.5) * 50),
+ z+((Math.random()-0.5) * 25)
+ );
+
+ //-- Particle color from pixel
+
+ var r = Math.round(pix[i]);
+ var g = Math.round(pix[i+1]);
+ var b = Math.round(pix[i+2]);
+
+
+ //-- Big particle
+
+ if(density>=2 && Math.abs(y)-1==0 && Math.random() * 1000 < 200) {
+ particlesBig.vertices.push(particle);
+ colorsBig[nbBig] = new THREE.Color("rgb("+r+", "+g+", "+b+")");
+ nbBig++;
+
+ //-- Small particle
+
+ } else if(density<4 || (Math.random() * 1000 < 400-(density*2))) {
+ particles.vertices.push(particle);
+ obj.colors[nb] = new THREE.Color("rgb("+r+", "+g+", "+b+")");
+ nb++;
+ }
+ };
+ }
+ }
+
+ //-- Create small particles milkyway
+
+ particles.colors = obj.colors;
+
+ var particleMaterial = new THREE.PointsMaterial({
+ map: Ed3d.textures.flare_yellow,
+ transparent: true,
+ size: 64,
+ vertexColors: THREE.VertexColors,
+ blending: THREE.AdditiveBlending,
+ depthTest: true,
+ depthWrite: false
+ });
+
+ var points = new THREE.Points(particles, particleMaterial);
+ points.sortParticles = true;
+ particles.center();
+
+ obj.milkyway[0] = points;
+ obj.milkyway[0].scale.set(20,20,20);
+
+ obj.obj.add(points);
+
+
+ //-- Create big particles milkyway
+
+ particlesBig.colors = colorsBig;
+
+ var particleMaterialBig = new THREE.PointsMaterial({
+ map: Ed3d.textures.flare_yellow,
+ transparent: true,
+ vertexColors: THREE.VertexColors,
+ size: 16,
+ blending: THREE.AdditiveBlending,
+ depthTest: true,
+ depthWrite: false
+ });
+
+ var pointsBig = new THREE.Points(particlesBig, particleMaterialBig);
+ pointsBig.sortParticles = true;
+ particlesBig.center();
+
+ obj.milkyway[1] = pointsBig;
+ obj.milkyway[1].scale.set(20,20,20);
+
+ obj.obj.add(pointsBig);
+ }
+
+}
diff --git a/js/components/grid.class.js b/js/components/grid.class.js
new file mode 100644
index 0000000..b58cc27
--- /dev/null
+++ b/js/components/grid.class.js
@@ -0,0 +1,192 @@
+
+var Grid = {
+
+ 'obj' : null,
+ 'size' : null,
+
+ 'textShapes' : null,
+ 'textGeo' : null,
+
+ 'coordTxt' : null,
+
+ 'minDistView' : null,
+
+ 'visible' : true,
+
+ 'fixed' : false,
+
+ /**
+ * Create 2 base grid scaled on Elite: Dangerous grid
+ */
+
+ 'init' : function(size, color, minDistView) {
+
+ this.size = size;
+
+ this.obj = new THREE.GridHelper(1000000, size);
+ this.obj.setColors(color, color);
+ this.obj.minDistView = minDistView;
+
+ scene.add(this.obj);
+
+ this.obj.customUpdateCallback = this.addCoords;
+
+
+ return this;
+ },
+
+ /**
+ * Create 2 base grid scaled on Elite: Dangerous grid
+ */
+
+ 'infos' : function(step, color, minDistView) {
+
+ var size = 50000;
+ if(step== undefined) step = 10000;
+ this.fixed = true;
+
+ //-- Add global grid
+
+ var geometry = new THREE.Geometry();
+ var material = new THREE.LineBasicMaterial( {
+ color: 0x555555,
+ transparent: true,
+ opacity: 0.2,
+ blending: THREE.AdditiveBlending,
+ depthWrite: false
+ } );
+
+ for ( var i = - size; i <= size; i += step ) {
+
+ geometry.vertices.push( new THREE.Vector3( - size, 0, i ) );
+ geometry.vertices.push( new THREE.Vector3( size, 0, i ) );
+
+ geometry.vertices.push( new THREE.Vector3( i, 0, - size ) );
+ geometry.vertices.push( new THREE.Vector3( i, 0, size ) );
+
+ }
+
+ this.obj = new THREE.LineSegments( geometry, material );
+ this.obj.position.set(0,0,-20000);
+
+ //-- Add quadrant
+
+ var quadrant = new THREE.Geometry();
+ var material = new THREE.LineBasicMaterial( {
+ color: 0x888888,
+ transparent: true,
+ opacity: 0.5,
+ blending: THREE.AdditiveBlending,
+ depthWrite: false
+ } );
+
+ quadrant.vertices.push( new THREE.Vector3( - size, 0, 20000 ) );
+ quadrant.vertices.push( new THREE.Vector3( size, 0, 20000 ) );
+
+ quadrant.vertices.push( new THREE.Vector3( 0, 0, - size ) );
+ quadrant.vertices.push( new THREE.Vector3( 0, 0, size ) );
+ var quadrantL = new THREE.LineSegments( quadrant, material );
+
+
+ this.obj.add(quadrantL);
+
+
+ //-- Add grid to the scene
+
+ scene.add(this.obj);
+
+ return this;
+ },
+
+ 'addCoords' : function() {
+
+ var textShow = '0 : 0 : 0';
+ var options = {
+ 'font': 'helvetiker',
+ 'weight': 'normal',
+ 'style': 'normal',
+ 'size': this.size/20,
+ 'curveSegments': 10
+ };
+
+ if(this.coordGrid != null) {
+
+ if(
+ Math.abs(camera.position.y-this.obj.position.y)>this.size*10
+ || Math.abs(camera.position.y-this.obj.position.y) < this.obj.minDistView
+ ) {
+ this.coordGrid.visible = false;
+ return;
+ }
+ this.coordGrid.visible = true;
+
+ var posX = Math.ceil(controls.target.x/this.size)*this.size;
+ var posZ = Math.ceil(controls.target.z/this.size)*this.size;
+
+ var textCoords = posX+' : '+this.obj.position.y+' : '+(-posZ);
+
+ //-- If same coords as previously, return.
+ if(this.coordTxt == textCoords) return;
+ this.coordTxt = textCoords;
+
+ //-- Generate a new text shape
+
+ this.textShapes = THREE.FontUtils.generateShapes( this.coordTxt, options );
+ this.textGeo.dispose();
+ this.textGeo = new THREE.ShapeGeometry(this.textShapes);
+
+ var center = this.textGeo.center();
+ this.coordGrid.position.set(center.x+posX-(this.size/100), this.obj.position.y, center.z+posZ+(this.size/30));
+
+
+ this.coordGrid.geometry = this.textGeo;
+ this.coordGrid.geometry.needsUpdate = true;
+
+ } else {
+
+ this.textShapes = THREE.FontUtils.generateShapes(textShow, options);
+ this.textGeo = new THREE.ShapeGeometry(this.textShapes);
+ this.coordGrid = new THREE.Mesh(this.textGeo, Ed3d.material.darkblue);
+ this.coordGrid.position.set(this.obj.position.x, this.obj.position.y, this.obj.position.z);
+ this.coordGrid.rotation.x = -Math.PI / 2;
+
+ scene.add(this.coordGrid);
+
+ }
+
+ },
+
+ /**
+ * Toggle grid view
+ */
+ 'toggleGrid' : function() {
+
+ this.visible = !this.visible;
+
+ if(this.size < 10000 && isFarView) return;
+ this.obj.visible = this.visible;
+
+ },
+
+ /**
+ * Show grid
+ */
+ 'show' : function() {
+
+ if(!this.visible) return;
+
+ this.obj.visible = true;
+
+ },
+
+ /**
+ * Hide grid
+ */
+ 'hide' : function() {
+
+ this.obj.visible = false;
+
+ }
+
+}
+
diff --git a/js/components/heat.class.js b/js/components/heat.class.js
new file mode 100644
index 0000000..037f48b
--- /dev/null
+++ b/js/components/heat.class.js
@@ -0,0 +1,125 @@
+
+var Heatmap = {
+
+ 'obj' : null,
+
+
+ 'create' : function(values) {
+
+ var PARTICLE_SIZE = 5000;
+ var geometry = new THREE.BufferGeometry;
+
+ var countItem = values.length*100;
+ var positions = new Float32Array( countItem * 3 );
+
+
+ var texloader = new THREE.TextureLoader();
+ var texSquare = texloader.load(Ed3d.basePath + "textures/lensflare/square.png");
+
+ var particleMaterial = new THREE.PointsMaterial({
+ map: texSquare,
+ vertexColors: THREE.VertexColors,
+ size: 800,
+ fog: false,
+ blending: THREE.AdditiveBlending,
+ transparent: true,
+ depthTest: true,
+ depthWrite: false
+ });
+
+ var colors = new Float32Array( countItem * 3 );
+ var color = new THREE.Color();
+
+
+ var heatColor = [
+ [63, 152, 180],
+ [107, 196, 165],
+ [165, 219, 163],
+ [217, 239, 152],
+ [247, 253, 177],
+ [254, 245, 176],
+ [253, 213, 128],
+ [253, 169, 94],
+ [245, 115, 69],
+ [222, 73, 73]
+ ];
+
+ var count = 0;
+ var grounpSize = 5;
+
+
+ $.each(values, function(i, prop) {
+
+
+
+ positions[ count ] = prop.x;
+ positions[ count + 1 ] = 0;
+ positions[ count + 2 ] = -prop.z;
+
+ var sizeColor = Math.round(prop.count/grounpSize);
+
+ if(sizeColor>heatColor.length-1) sizeColor = heatColor.length-1;
+
+
+ colors[ count ] = heatColor[sizeColor][0]/255;
+ colors[ count + 1 ] = heatColor[sizeColor][1]/255;
+ colors[ count + 2 ] = heatColor[sizeColor][2]/255;
+
+ count += 3;
+
+
+ //-- TEST WITH Particle MORE !!!!
+ /* var height = Math.abs(prop.y[1] - prop.y[0])/2;
+ if(height>100) height = 100;
+ for (var i = 1; i heatColor.length-1) sizeColor = heatColor.length-1;
+
+
+ colors[ count ] = heatColor[sizeColor][0]/255;
+ colors[ count + 1 ] = heatColor[sizeColor][1]/255;
+ colors[ count + 2 ] = heatColor[sizeColor][2]/255;
+
+ count += 3;
+
+ }*/
+
+ //-- TEST WITH CUBE
+
+
+ var height = Math.abs(prop.y[1] - prop.y[0])*2;
+ if(height<100) height = 100;
+
+ var geometry = new THREE.BoxBufferGeometry( 170, height, 170 );
+ var material = new THREE.MeshLambertMaterial( {color: "rgb("+heatColor[sizeColor][0]+", "+heatColor[sizeColor][1]+", "+heatColor[sizeColor][2]+")"} );
+
+
+ var cube = new THREE.Mesh( geometry, material );
+ cube.position.set(prop.x, height/2, -prop.z);
+
+ scene.add( cube );
+
+ //-- TEST WITH CUBE
+
+
+ });
+
+
+
+
+ geometry.addAttribute( 'position', new THREE.BufferAttribute( positions, 3 ) );
+ geometry.addAttribute( 'color', new THREE.BufferAttribute( colors, 3 ) );
+
+
+ var particles = new THREE.Points(geometry, particleMaterial);
+
+ //scene.add(particles);
+ }
+
+}
diff --git a/js/components/hud.class.js b/js/components/hud.class.js
new file mode 100644
index 0000000..ddc037d
--- /dev/null
+++ b/js/components/hud.class.js
@@ -0,0 +1,598 @@
+
+var HUD = {
+
+ 'container' : null,
+
+ /**
+ *
+ */
+ 'init' : function() {
+
+ Loader.update('Init HUD');
+ this.initHudAction();
+ this.initControls();
+
+ },
+
+ /**
+ *
+ */
+ 'create' : function(container) {
+
+ this.container = container;
+
+ if(!$('#'+this.container+' #controls').length && Ed3d.withOptionsPanel == true) {
+
+ $('#'+this.container).append(
+ ' '
+ );
+ this.createSubOptions();
+
+ //-- Optionnal button to go fuulscreen
+
+ if(Ed3d.withFullscreenToggle) {
+ $( "" )
+ .attr("id", "tog-fullscreen" )
+ .html('Fullscreen')
+ .click(function() {
+ $('#'+container).toggleClass('map-fullscreen');
+ refresh3dMapSize();
+ })
+ .prependTo( "#controls" );
+ }
+
+
+ }
+
+ if(!Ed3d.withHudPanel) return;
+
+ $('#'+this.container).append('');
+ $('#hud').append(
+ ''+
+ '
Infos
'+
+ ' Dist. Sol
'+
+ '
'+
+ '
'+
+ '
'+
+ '
'+
+ ' '+
+ '
Search
'+
+ ' '+
+ ' '+
+ ' '+
+ '
'+
+ ''
+ );
+
+ var addClass = (Ed3d.popupDetail ? 'class="popup-detail"' : '');
+ $('#'+this.container).append('');
+
+ },
+
+ /**
+ * Create option panel
+ */
+ 'createSubOptions' : function() {
+
+ //-- Toggle milky way
+ $( "" )
+ .addClass( "sub-opt active" )
+ .html('Toggle Milky Way')
+ .click(function() {
+ var state = Galaxy.milkyway[0].visible;
+ Galaxy.milkyway[0].visible = !state;
+ Galaxy.milkyway[1].visible = !state;
+ Galaxy.milkyway2D.visible = !state;
+ $(this).toggleClass('active');
+ })
+ .appendTo( "#options" );
+
+ //-- Toggle Grid
+ $( "" )
+ .addClass( "sub-opt active" )
+ .html('Toggle grid')
+ .click(function() {
+ Ed3d.grid1H.toggleGrid();
+ Ed3d.grid1K.toggleGrid();
+ Ed3d.grid1XL.toggleGrid();
+ $(this).toggleClass('active');
+ })
+ .appendTo( "#options" );
+
+ },
+
+ /**
+ * Controls init for camera views
+ */
+ 'initControls' : function() {
+
+ $('#controls a').click(function(e) {
+
+ if($(this).hasClass('view')) {
+ $('#controls a.view').removeClass('selected')
+ $(this).addClass('selected');
+ }
+
+ var view = $(this).data('view');
+
+
+ switch(view) {
+
+ case 'top':
+ Ed3d.isTopView = true;
+ var moveFrom = {x: camera.position.x, y: camera.position.y , z: camera.position.z};
+ var moveCoords = {x: controls.target.x, y: controls.target.y+500, z: controls.target.z};
+ HUD.moveCamera(moveFrom,moveCoords);
+ break;
+
+ case '3d':
+ Ed3d.isTopView = false;
+ var moveFrom = {x: camera.position.x, y: camera.position.y , z: camera.position.z};
+ var moveCoords = {x: controls.target.x-100, y: controls.target.y+500, z: controls.target.z+500};
+ HUD.moveCamera(moveFrom,moveCoords);
+ break;
+
+ case 'infos':
+ if(!Ed3d.showGalaxyInfos) {
+ Ed3d.showGalaxyInfos = true;
+ Galaxy.infosShow();
+ } else {
+ Ed3d.showGalaxyInfos = false;
+ Galaxy.infosHide();
+ }
+ $(this).toggleClass('selected');
+ break;
+
+ case 'options':
+ $('#options').toggle();
+ break;
+
+ }
+
+
+
+
+ });
+
+ },
+
+ /**
+ * Move camera to a target
+ */
+ 'moveCamera' : function(from, to) {
+
+ Ed3d.tween = new TWEEN.Tween(from, {override:true}).to(to, 800)
+ .start()
+ .onUpdate(function () {
+ camera.position.set(from.x, from.y, from.z);
+ })
+ .onComplete(function () {
+ controls.update();
+ });
+
+ },
+
+ /**
+ *
+ */
+ 'initHudAction' : function() {
+
+ //-- Disable 3D controls when mouse hover the Hud
+ $( "canvas" ).hover(
+ function() {
+ controls.enabled = true;
+ }, function() {
+ controls.enabled = false;
+ }
+ );
+
+ //-- Disable 3D controls when mouse hover the Hud
+ $( "#hud" ).hover(
+ function() {
+ controls.enabled = false;
+ }, function() {
+ controls.enabled = true;
+ }
+ );
+ $( "#systemDetails" ).hide();
+
+ //-- Add Count filters
+ $('.map_filter').each(function(e) {
+ var idCat = $(this).data('filter');
+ var count = Ed3d.catObjs[idCat].length;
+ if(count>1) $(this).append(' ('+count+')');
+ });
+
+ //-- Add map filters
+ $('.map_filter').click(function(e) {
+ e.preventDefault();
+ var idCat = $(this).data('filter');
+ var active = $(this).data('active');
+ active = (Math.abs(active-1));
+
+ //------------------------------------------------------------------------
+ //-- Single item by once
+
+ if(!Ed3d.hudMultipleSelect) {
+
+ $('.map_filter').addClass('disabled');
+
+ //-- Toggle systems particles
+ $(System.particleGeo.vertices).each(function(index, point) {
+ point.visible = 0;
+ point.filtered = 0;
+ System.particleGeo.colors[index] = new THREE.Color('#111111');
+ active = 1;
+ });
+
+
+ //-- Toggle routes
+ if(Ed3d.catObjsRoutes.length>0)
+ $(Ed3d.catObjsRoutes).each(function(indexCat, listGrpRoutes) {
+ if(listGrpRoutes != undefined)
+ $(listGrpRoutes).each(function(key, indexRoute) {
+ scene.getObjectByName( indexRoute ).visible = false;
+ if(scene.getObjectByName( indexRoute+'-first' ) != undefined)
+ scene.getObjectByName( indexRoute+'-first' ).visible = false;
+ if(scene.getObjectByName( indexRoute+'-last' ) != undefined)
+ scene.getObjectByName( indexRoute+'-last' ).visible = false;
+ });
+ });
+
+ }
+
+ //------------------------------------------------------------------------
+ //-- multiple select
+
+ var center = null;
+ var nbPoint = 0;
+ var pointFar = null;
+
+ //-- Toggle routes
+
+ if(Ed3d.catObjsRoutes.length>0)
+ $(Ed3d.catObjsRoutes[idCat]).each(function(key, indexRoute) {
+ var isVisible = scene.getObjectByName( indexRoute ).visible;
+ if(isVisible == undefined) isVisible = true;
+ isVisible = (isVisible ? false : true);
+ scene.getObjectByName( indexRoute ).visible = isVisible;
+ if(scene.getObjectByName( indexRoute+'-first' ) != undefined)
+ scene.getObjectByName( indexRoute+'-first' ).visible = isVisible;
+ if(scene.getObjectByName( indexRoute+'-last' ) != undefined)
+ scene.getObjectByName( indexRoute+'-last' ).visible = isVisible;
+ });
+
+ //-- Toggle systems particles
+
+ $(Ed3d.catObjs[idCat]).each(function(key, indexPoint) {
+
+ obj = System.particleGeo.vertices[indexPoint];
+
+ System.particleGeo.colors[indexPoint] = (active==1)
+ ? obj.color
+ : new THREE.Color('#111111');
+
+ obj.visible = (active==1);
+ obj.filtered = (active==1);
+
+ System.particleGeo.colorsNeedUpdate = true;
+
+ //-- Sum coords to detect the center & detect the most far point
+ if(center == null) {
+ center = new THREE.Vector3(obj.x, obj.y, obj.z);
+ pointFar = new THREE.Vector3(obj.x, obj.y, obj.z);
+ } else {
+ center.set(
+ (center.x + obj.x),
+ (center.y + obj.y),
+ (center.z + obj.z)
+ );
+ if(
+ (Math.abs(pointFar.x) - Math.abs(obj.x))+
+ (Math.abs(pointFar.y) - Math.abs(obj.y))+
+ (Math.abs(pointFar.z) - Math.abs(obj.z)) < 0
+ ) {
+ pointFar.set(obj.x, obj.y, obj.z);
+ }
+ }
+ nbPoint++;
+
+ });
+
+ if(nbPoint==0) return;
+
+ //------------------------------------------------------------------------
+ //-- Calc center of all selected points
+
+ center.set(
+ Math.round(center.x/nbPoint),
+ Math.round(center.y/nbPoint),
+ -Math.round(center.z/nbPoint)
+ );
+
+ $(this).data('active',active);
+ $(this).toggleClass('disabled');
+
+ //-- If current selection is no more visible, disable active selection
+ if(Action.oldSel != null && !Action.oldSel.visible) Action.disableSelection();
+
+ //-- Calc max distance from center of selection
+ var distance = pointFar.distanceTo( center )+200;
+
+ //-- Set new camera & target position
+ //Ed3d.playerPos = [center.x,center.y,center.z];
+ //Ed3d.cameraPos = [
+ // center.x + (Math.floor((Math.random() * 100) + 1)-50), //-- Add a small rotation effect
+ // center.y + distance,
+ // center.z - distance
+ //];
+
+ //Action.moveInitalPosition();
+ });
+
+
+ //-- Add map link
+ $('.map_link').click(function(e) {
+
+ e.preventDefault();
+ var elId = $(this).data('route');
+ Action.moveToObj(routes[elId]);
+ });
+
+ $('.map_link span').click(function(e) {
+
+ e.preventDefault();
+
+ var elId = $(this).parent().data('route');
+ routes[elId].visible = !routes[elId].visible;
+ });
+
+ },
+
+
+ /**
+ * Init filter list
+ */
+
+ 'initFilters' : function(categories) {
+
+ Loader.update('HUD Filter...');
+
+ var grpNb = 1;
+ $.each(categories, function(typeFilter, values) {
+
+ if(typeof values === "object" ) {
+
+ var groupId = 'group_'+grpNb;
+ var nbFilters = values.length;
+ var count = 0;
+ var visible = true;
+
+ $('#filters').append(''+typeFilter+'
');
+ $('#filters').append('');
+
+ $.each(values, function(key, val) {
+
+ visible = true;
+
+ //-- Manage view limit if activated
+
+ if(Ed3d.categoryAutoCollapseSize !== false) {
+ count++;
+ if(count>Ed3d.categoryAutoCollapseSize) visible = false;
+ }
+
+ //-- Add filter
+
+ HUD.addFilter(groupId, key, val, visible);
+ Ed3d.catObjs[key] = [];
+
+ });
+
+ grpNb++;
+
+ //-- If more childs than 'categoryAutoCollapseSize' value
+ //-- add the button to toggle items
+
+ if(visible==false) {
+
+ $('#'+groupId).append(
+ ''+
+ '+ See more' +
+ ''
+ ).click(function(){
+ HUD.expandFilters(groupId);
+ });
+
+ }
+ }
+
+ });
+
+
+ },
+
+ /**
+ * Expand filter
+ */
+
+ 'expandFilters' : function(groupId) {
+
+ $('#'+groupId)
+ .addClass('open');
+
+ $('#hud').addClass('enlarge');
+
+
+ },
+
+ /**
+ * Remove filters list
+ */
+
+ 'removeFilters' : function() {
+
+ $('#hud #filters').html('');
+
+ },
+
+
+ /**
+ *
+ */
+ 'addFilter' : function(groupId, idCat, val, visible) {
+
+ //-- Add material, if custom color defined, use it
+ var back = '#fff';
+ var addClass = '';
+
+ if(val.color != undefined) {
+ Ed3d.addCustomMaterial(idCat, val.color);
+ back = '#'+val.color;
+ }
+
+ if(!visible) {
+ addClass += ' hidden';
+ }
+
+ //-- Add html link
+ $('#'+groupId).append(
+ ''+
+ ' ' + val.name +
+ ''
+ );
+ },
+
+ /**
+ *
+ */
+ 'openHudDetails' : function() {
+ $('#hud').hide();
+ $('#systemDetails').show().hover(
+ function() {
+ controls.enabled = false;
+ }, function() {
+ controls.enabled = true;
+ }
+ );
+ },
+ /**
+ *
+ */
+ 'closeHudDetails' : function() {
+ $('#hud').show();
+ $('#systemDetails').hide();
+ },
+
+ /**
+ * Create a Line route
+ */
+ 'setRoute' : function(idRoute, nameR) {
+ $('#routes').append(' ' + nameR + '');
+ },
+
+
+
+ /**
+ *
+ */
+
+ 'setInfoPanel' : function(index, point) {
+
+ $('#systemDetails').html(
+ ''+point.name+'
'+
+ ''+
+ ' '+point.x+''+point.y+''+(-point.z)+'
'+
+ ' '+
+ ''+
+ (point.infos != undefined ? ''+point.infos+'
' : '')+
+ ''+
+ '
'
+ );
+
+ //-- Add navigation
+
+ $('', {'html': '<'})
+ .click(function(){Action.moveNextPrev(index-1, -1);})
+ .appendTo("#nav");
+
+ $('', {'html': 'X'})
+ .click(function(){HUD.closeHudDetails();})
+ .appendTo("#nav");
+
+ $('', {'html': '>'})
+ .click(function(){Action.moveNextPrev(index+1, 1);})
+ .appendTo("#nav");
+
+ },
+
+
+ /**
+ * Add Shape text
+ */
+
+ 'addText' : function(id, textShow, x, y, z, size, addToObj, isPoint) {
+
+ if(addToObj == undefined) addToObj = scene;
+ if(isPoint == undefined) isPoint = false;
+
+ var textShapes = THREE.FontUtils.generateShapes(textShow, {
+ 'font': 'helvetiker',
+ 'weight': 'normal',
+ 'style': 'normal',
+ 'size': size,
+ 'curveSegments': 100
+ });
+
+ var textGeo = new THREE.ShapeGeometry(textShapes);
+
+ if(Ed3d.textSel[id] == undefined) {
+ var textMesh = new THREE.Mesh(textGeo, new THREE.MeshBasicMaterial({
+ color: 0xffffff
+ }));
+ } else {
+ var textMesh = Ed3d.textSel[id];
+ }
+
+ textMesh.geometry = textGeo;
+ textMesh.geometry.needsUpdate = true;
+
+ if(isPoint) {
+ textMesh.position.set(addToObj.x, addToObj.y, addToObj.z);
+ textMesh.name = id;
+ scene.add(textMesh);
+ } else {
+ textMesh.position.set(x, y, z);
+ addToObj.add(textMesh);
+ }
+
+ Ed3d.textSel[id] = textMesh;
+
+ },
+
+ /**
+ * Add Shape text
+ */
+
+ 'rotateText' : function(id) {
+
+ //y = -Math.abs(y);
+
+ if(Ed3d.textSel[id] != undefined)
+ if(Ed3d.isTopView) {
+ Ed3d.textSel[id].rotation.set(-Math.PI/2,0,0);
+ } else {
+ Ed3d.textSel[id].rotation.x = 0;
+ Ed3d.textSel[id].rotation.y = camera.rotation.y;
+ Ed3d.textSel[id].rotation.z = 0;
+ }
+
+ }
+}
\ No newline at end of file
diff --git a/js/components/icon.class.js b/js/components/icon.class.js
new file mode 100644
index 0000000..629c750
--- /dev/null
+++ b/js/components/icon.class.js
@@ -0,0 +1,6 @@
+
+var Ico = {
+
+ 'cog' : ''
+
+}
\ No newline at end of file
diff --git a/js/components/route.class.js b/js/components/route.class.js
new file mode 100644
index 0000000..62c6943
--- /dev/null
+++ b/js/components/route.class.js
@@ -0,0 +1,258 @@
+
+var Route = {
+
+ //-- Var to enable routes
+ 'active' : false,
+
+ //-- Init list coords
+ 'systems' : [],
+
+ //-- Route list
+ 'list' : [],
+
+ /**
+ * Initialize the systems list for coordinates check
+ *
+ * @param {Int} Unique ID for the route
+ * @param {JSON} A JSon list of points
+ */
+
+ 'initRoute' : function(idRoute, route) {
+
+ Route.active = true;
+
+ $.each(route.points, function(key, val) {
+ Route.systems[val.s] = false;
+ if(val.coords != undefined) {
+ val.name = val.s;
+ System.create(val);
+ }
+ });
+
+ },
+
+ /**
+ * Remove routes
+ */
+
+ 'remove' : function() {
+
+ $(Ed3d.catObjsRoutes).each(function(indexCat, listGrpRoutes) {
+ if(listGrpRoutes != undefined)
+
+ $(listGrpRoutes).each(function(key, indexRoute) {
+ scene.remove(scene.getObjectByName( indexRoute ));
+ if(scene.getObjectByName( indexRoute+'-first' ) != undefined)
+ scene.remove(scene.getObjectByName( indexRoute+'-first' ));
+ if(scene.getObjectByName( indexRoute+'-last' ) != undefined)
+ scene.remove(scene.getObjectByName( indexRoute+'-last' ));
+ });
+ });
+
+
+ },
+
+ /**
+ * Create a Line route
+ *
+ * @param {Int} Unique ID for the route
+ * @param {JSON} A JSon list of points
+ */
+
+ 'createRoute' : function(idRoute, route) {
+
+ var geometryL = new THREE.Geometry();
+ var nameR = '';
+ var first = null;
+ var last = null;
+ var color = Ed3d.material.orange;
+ var colorLine = Ed3d.material.line;
+ var circle = true;
+
+ var hideLast = (route.hideLast !== undefined && route.hideLast);
+
+ //console.log(route);
+
+ if(route.cat !== undefined && route.cat[0] != undefined && Ed3d.colors[route.cat[0]] != undefined) {
+ color = new THREE.MeshBasicMaterial({
+ color: Ed3d.colors[route.cat[0]]
+ });
+ colorLine = new THREE.MeshBasicMaterial({
+ color: Ed3d.colors[route.cat[0]]
+ });
+ } else {
+ color = Ed3d.material.orange;
+ }
+
+ $.each(route.points, function(key2, val) {
+
+ if(Route.systems[val.s] !== false) {
+
+ var c = Route.systems[val.s];
+
+ if(first==null) first = [c[0], c[1], c[2]];
+ last = [c[0], c[1], c[2]];
+
+ //-- Add line point
+ geometryL.vertices.push(
+ new THREE.Vector3(c[0], c[1], c[2])
+ );
+ //Route.addPoint(c[0], c[1], c[2]);
+
+
+ } else {
+
+ console.log("Missing point: "+val.s);
+ }
+
+ });
+
+ //-- Add lines to scene
+ routes[idRoute] = new THREE.Line(geometryL, colorLine);
+
+ //-- Add object for start & end
+
+ if(route.circle != undefined) {
+ circle = route.circle
+ } else {
+ circle = true
+ }
+
+ if (circle != false) {
+ if(first!==null) this.addCircle('route-'+idRoute+'-first', first, color, 7);
+ if(!hideLast && last!==null) this.addCircle('route-'+idRoute+'-last', last, color, 3);
+ }
+
+ routes[idRoute].name = 'route-'+idRoute;
+
+ scene.add(routes[idRoute]);
+
+
+ if(route.cat !== undefined) {
+ $.each(route.cat, function(keyArr, idCat) {
+ if(Ed3d.catObjsRoutes[idCat] == undefined)
+ Ed3d.catObjsRoutes[idCat] = [];
+ Ed3d.catObjsRoutes[idCat].push(routes[idRoute].name);
+ });
+ }
+
+ },
+
+ 'addCircle' : function(id, point, material, size) {
+
+ var cursor = new THREE.Object3D;
+
+ //-- Ring around the system
+ var geometryL = new THREE.TorusGeometry( size, 0.4, 3, 15 );
+
+ var selection = new THREE.Mesh(geometryL, material);
+ selection.rotation.x = Math.PI / 2;
+
+ cursor.add(selection);
+
+ cursor.position.set(point[0], point[1], point[2]);
+
+ cursor.scale.set(15,15,15);
+
+ cursor.name = id;
+ scene.add(cursor);
+
+ //-- Add to cursor list for scale effets
+ Action.cursor[cursor.id] = cursor;
+
+ },
+
+ 'addPoint' : function(x, y, z, name) {
+
+ /*console.log('Add point route');*/
+
+ var cursor = new THREE.Object3D;
+
+ //-- Ring around the system
+ var geometryL = new THREE.TorusGeometry( 12, 0.4, 3, 30 );
+
+ var selection = new THREE.Mesh(geometryL, Ed3d.material.selected);
+ selection.rotation.x = Math.PI / 2;
+
+ cursor.add(selection);
+
+ //-- Create a cone on the selection
+ var geometryCone = new THREE.CylinderGeometry(0, 5, 16, 4, 1, false);
+ var cone = new THREE.Mesh(geometryCone, Ed3d.material.selected);
+ cone.position.set(0, 20, 0);
+ cone.rotation.x = Math.PI;
+ cursor.add(cone);
+
+ //-- Inner cone
+ var geometryConeInner = new THREE.CylinderGeometry(0, 3.6, 16, 4, 1, false);
+ var coneInner = new THREE.Mesh(geometryConeInner, Ed3d.material.black);
+ coneInner.position.set(0, 20.2, 0);
+ coneInner.rotation.x = Math.PI;
+ cursor.add(coneInner);
+
+
+ cursor.position.set(x, y, z);
+
+ cursor.scale.set(30,30,30);
+
+ scene.add(cursor);
+
+ },
+
+ 'newRoute' : function(name) {
+ var routes = localStorage.getItem("routes");
+ if(routes == undefined) {
+ var routes = [];
+ }
+
+ var myRoute = {
+ title: "Test route",
+ points: []
+ }
+
+ routes.push(myRoute);
+ routes.push(myRoute);
+ routes.push(myRoute);
+
+ localStorage.setItem("routes",JSON.stringify(routes));
+ },
+
+ 'addPointToRoute' : function(x,y,z,system,label) {
+
+ idRoute = 1;
+
+ console.log('add point');
+
+ var routes = JSON.parse(localStorage.getItem("routes"));
+ if(routes[idRoute] == undefined) return false;
+
+ var point = {
+ "coords": {"x":x, "y":y, "z":z }
+ }
+ if(system==undefined) system = Math.floor(Date.now() / 1000);
+
+ if(system != undefined) point.s = system;
+ if(label != undefined) point.label = label;
+
+ routes[idRoute].points.push(point);
+
+ localStorage.setItem("routes",JSON.stringify(routes));
+
+
+
+ this.drawRoute(1);
+ },
+
+ 'drawRoute': function(idRoute) {
+
+ var route = JSON.parse(localStorage.getItem("routes"))[idRoute];
+ if(route == undefined) return false;
+
+ this.initRoute(0,route);
+ this.createRoute(0,route);
+
+ },
+
+
+
+}
diff --git a/js/components/system.class.js b/js/components/system.class.js
new file mode 100644
index 0000000..fc46644
--- /dev/null
+++ b/js/components/system.class.js
@@ -0,0 +1,182 @@
+
+var System = {
+
+ 'particle' : null,
+ 'particleGeo' : null,
+ 'particleColor' : [],
+ 'particleInfos' : [],
+ 'count' : 0,
+ 'scaleSize' : 64,
+
+ /**
+ * Add a system in galaxy
+ *
+ * @param {object} val System properties (x, y, z, name are mandatory)
+ * @param {string} withSolid Add a solid sphere (default: false)
+ */
+
+ 'create' : function(val, withSolid) {
+
+ if(withSolid==undefined) withSolid = false;
+
+ if(val.coords==undefined) return false;
+
+ var x = parseInt(val.coords.x);
+ var y = parseInt(val.coords.y);
+ var z = -parseInt(val.coords.z); //-- Revert Z coord
+
+ //--------------------------------------------------------------------------
+ //-- Particle for near and far view
+
+ var colors = [];
+ if(this.particleGeo !== null) {
+
+ //-- If system with info already registered, concat datas
+ var idSys = x+'_'+y+'_'+z;
+ if(val.infos != undefined && this.particleInfos[idSys]) {
+ var indexParticle = this.particleInfos[idSys];
+ this.particleGeo.vertices[indexParticle].infos += val.infos;
+ if(val.cat != undefined) Ed3d.addObjToCategories(indexParticle,val.cat);
+ return;
+ }
+
+ var particle = new THREE.Vector3(x, y, z);
+
+ //-- Get point color
+
+ if(val.cat != undefined && val.cat[0] != undefined && Ed3d.colors[val.cat[0]] != undefined) {
+ this.particleColor[this.count] = Ed3d.colors[val.cat[0]];
+ } else {
+ this.particleColor[this.count] = new THREE.Color(Ed3d.systemColor);
+ }
+
+ //-- If system got some categories, add it to cat list and save his main color
+
+ if(val.cat != undefined) {
+ Ed3d.addObjToCategories(this.count,val.cat);
+ particle.color = this.particleColor[this.count];
+ }
+
+ //-- Attach name and set point as clickable
+
+ particle.clickable = true;
+ particle.visible = true;
+ particle.name = val.name;
+ if(val.infos != undefined) {
+ particle.infos = val.infos;
+ this.particleInfos[idSys] = this.count;
+ }
+ if(val.url != undefined) {
+ particle.url = val.url;
+ }
+
+ this.particleGeo.vertices.push(particle);
+
+ this.count++;
+ }
+
+ //--------------------------------------------------------------------------
+ //-- Check if we have to add coords for a route
+
+ if(Route.active == true) {
+
+ if(Route.systems[val.name] != undefined) {
+ Route.systems[val.name] = [x,y,z]
+ }
+
+ }
+
+ //--------------------------------------------------------------------------
+ //-- Build a sphere if needed
+
+ if(withSolid) {
+
+ //-- Add glow sprite from first cat color if defined, else take white glow
+
+ var mat = Ed3d.material.glow_1;
+
+ var sprite = new THREE.Sprite( mat );
+ sprite.position.set(x, y, z);
+ sprite.scale.set(50, 50, 1.0);
+ scene.add(sprite); // this centers the glow at the mesh
+
+ //-- Sphere
+
+ var geometry = new THREE.SphereGeometry(2, 10, 10);
+
+ var sphere = new THREE.Mesh(geometry, Ed3d.material.white);
+
+ sphere.position.set(x, y, z);
+
+ sphere.name = val.name;
+
+ sphere.clickable = true;
+ sphere.idsprite = sprite.id;
+ scene.add(sphere);
+
+ return sphere;
+ }
+
+ },
+
+
+ /**
+ * Init the galaxy particle geometry
+ */
+
+ 'initParticleSystem' : function () {
+ this.particleGeo = new THREE.Geometry;
+ },
+
+ /**
+ * Create the particle system
+ */
+
+ 'endParticleSystem' : function () {
+
+ if(this.particleGeo == null) return;
+
+ this.particleGeo.colors = this.particleColor;
+
+ var particleMaterial = new THREE.PointsMaterial({
+ map: Ed3d.textures.flare_yellow,
+ vertexColors: THREE.VertexColors,
+ size: this.scaleSize,
+ fog: false,
+ blending: THREE.AdditiveBlending,
+ transparent: true,
+ depthTest: true,
+ depthWrite: false
+ });
+
+ this.particle = new THREE.Points(this.particleGeo, particleMaterial);
+
+ this.particle.sortParticles = true;
+ this.particle.clickable = true;
+
+ scene.add(this.particle);
+ },
+
+
+ /**
+ * Remove systems list
+ */
+
+ 'remove' : function() {
+
+ this.particleColor = [];
+ this.particleGeo = null;
+ this.count = 0;
+ scene.remove(this.particle);
+
+ },
+
+ /**
+ * Load Spectral system color
+ */
+
+ 'loadSpectral' : function(val) {
+
+ }
+
+}
diff --git a/js/ed3dmap.js b/js/ed3dmap.js
new file mode 100644
index 0000000..79aa895
--- /dev/null
+++ b/js/ed3dmap.js
@@ -0,0 +1,931 @@
+//--
+var camera;
+var controls;
+var scene;
+var light;
+var renderer;
+
+var raycaster;
+
+var composer;
+
+//-- Map Vars
+var container;
+var routes = [];
+var lensFlareSel;
+
+
+var Ed3d = {
+
+ 'container' : null,
+ 'basePath' : './',
+
+ 'jsonPath' : null,
+ 'jsonContainer' : null,
+ 'json' : null,
+
+ 'grid1H' : null,
+ 'grid1K' : null,
+ 'grid1XL' : null,
+
+ 'tween' : null,
+
+ 'globalView' : true,
+
+ //-- Fog density save
+ 'fogDensity' : null,
+
+ //-- Defined texts
+ 'textSel' : [],
+
+ //-- Object list by categories
+ 'catObjs' : [],
+ 'catObjsRoutes' : [],
+
+ //-- Materials
+ 'material' : {
+ 'Trd' : new THREE.MeshBasicMaterial({
+ color: 0xffffff
+ }),
+ 'line' : new THREE.LineBasicMaterial({
+ color: 0xcccccc
+ }),
+ 'white' : new THREE.MeshBasicMaterial({
+ color: 0xffffff
+ }),
+ 'orange' : new THREE.MeshBasicMaterial({
+ color: 0xFF9D00
+ }),
+ 'black' : new THREE.MeshBasicMaterial({
+ color: 0x010101
+ }),
+ 'lightblue' : new THREE.MeshBasicMaterial({
+ color: 0x0E7F88
+ }),
+ 'darkblue' : new THREE.MeshBasicMaterial({
+ color: 0x16292B
+ }),
+ 'selected' : new THREE.MeshPhongMaterial({
+ color: 0x0DFFFF
+ }),
+ 'grey' : new THREE.MeshPhongMaterial({
+ color: 0x7EA0A0
+ }),
+ 'transparent' : new THREE.MeshBasicMaterial({
+ color: 0x000000,
+ transparent: true,
+ opacity: 0
+ }),
+ 'glow_1' : null,
+ 'custom' : []
+ },
+
+ 'starSprite' : 'textures/lensflare/star_grey2.png',
+
+ 'colors' : [],
+ 'textures' : {},
+
+ //-- Default color for system sprite
+ 'systemColor' : '#eeeeee',
+
+ //-- HUD
+ 'withHudPanel' : false,
+ 'withOptionsPanel' : true,
+ 'hudMultipleSelect' : true,
+
+ //-- Systems
+ 'systems' : [],
+
+ //-- Starfield
+ 'starfield' : null,
+
+ //-- Start animation
+ 'startAnim' : true,
+
+ //-- Scale system effect
+ 'effectScaleSystem' : [10,800],
+
+ //-- Graphical Options
+ 'optDistObj' : 1500,
+
+ //-- Player position
+ 'playerPos' : [0, 0, 0],
+
+ //-- Initial camera position
+ 'cameraPos' : null,
+
+ //-- Active 2D top view
+ 'isTopView' : false,
+
+ //-- Show galaxy infos
+ 'showGalaxyInfos' : false,
+
+ //-- Show names near camera
+ 'showNameNear' : false,
+
+ //-- Popup mode for click on detal
+ 'popupDetail' : false,
+
+ //-- Objects
+ 'Action' : null,
+ 'Galaxy' : null,
+
+ //-- With button to toggle fullscreen
+ 'withFullscreenToggle' : false,
+
+ //-- Collapse subcategories (false: don't collapse)
+ 'categoryAutoCollapseSize' : false,
+
+ /**
+ * Init Ed3d map
+ *
+ */
+
+ 'init' : function(options) {
+
+ // Merge options with defaults Ed3d
+ var options = $.extend(Ed3d, options);
+
+ //-- Init 3D map container
+ $('#'+Ed3d.container).append('');
+
+
+ //-- Load dependencies
+ Loader.update('Load core files');
+
+ if(typeof isMinified !== 'undefined') return Ed3d.launchMap();
+
+ $.when(
+ $.getScript(Ed3d.basePath + "vendor/three-js/OrbitControls.js"),
+ $.getScript(Ed3d.basePath + "vendor/three-js/CSS3DRenderer.js"),
+ $.getScript(Ed3d.basePath + "vendor/three-js/Projector.js"),
+ $.getScript(Ed3d.basePath + "vendor/three-js/FontUtils.js"),
+ $.Deferred(function( deferred ){
+ $( deferred.resolve );
+ })
+ ).done(function(){
+ $.when(
+ $.getScript(Ed3d.basePath + "vendor/three-js/helvetiker_regular.typeface.js"),
+
+ $.getScript(Ed3d.basePath + "js/components/grid.class.js"),
+ $.getScript(Ed3d.basePath + "js/components/icon.class.js"),
+ $.getScript(Ed3d.basePath + "js/components/hud.class.js"),
+ $.getScript(Ed3d.basePath + "js/components/action.class.js"),
+ $.getScript(Ed3d.basePath + "js/components/route.class.js"),
+ $.getScript(Ed3d.basePath + "js/components/system.class.js"),
+ $.getScript(Ed3d.basePath + "js/components/galaxy.class.js"),
+ $.getScript(Ed3d.basePath + "js/components/heat.class.js"),
+
+ $.getScript(Ed3d.basePath + "vendor/tween-js/Tween.js"),
+
+ $.Deferred(function( deferred ){
+ $( deferred.resolve );
+ })
+ ).done(function() {
+ Loader.update('Done !');
+ Ed3d.launchMap();
+ if(typeof options.finished === "function") options.finished();
+ }).fail(function(jqXHR, textStatus, errorThrown){
+ console.log(errorThrown)
+ });
+ })
+ },
+
+ /**
+ * Init objects
+ */
+
+ 'initObjects' : function(options) {
+
+ //-- Init Object
+ this.Action = Action;
+ this.Galaxy = Galaxy;
+
+ },
+
+ /**
+ * Rebuild completely system list and filter (for new JSon content)
+ */
+
+ 'rebuild' : function(options) {
+
+ Loader.start();
+
+ // Remove System & HUD filters
+ this.destroy();
+
+ // Reload from JSon
+ if(this.jsonPath != null) Ed3d.loadDatasFromFile();
+ else if(this.jsonContainer != null) Ed3d.loadDatasFromContainer();
+
+ this.Action.moveInitalPosition();
+
+ Loader.stop();
+
+ },
+
+ /**
+ * Destroy the 3dmap
+ */
+
+ 'destroy' : function() {
+
+ Loader.start();
+
+ // Remove System & HUD filters
+ System.remove();
+ HUD.removeFilters();
+ Route.remove();
+ Galaxy.remove();
+
+ Loader.stop();
+
+ },
+
+ /**
+ * Launch
+ */
+
+ 'launchMap' : function() {
+
+ this.initObjects();
+
+ Loader.update('Textures');
+ Ed3d.loadTextures();
+
+ Loader.update('Launch scene');
+ Ed3d.initScene();
+
+ // Create grid
+
+ Ed3d.grid1H = $.extend({}, Grid.init(100, 0x111E23, 0), {});
+ Ed3d.grid1K = $.extend({}, Grid.init(1000, 0x22323A, 1000), {});
+ Ed3d.grid1XL = $.extend({}, Grid.infos(10000, 0x22323A, 10000), {});
+
+
+ // Add some scene enhancement
+ Ed3d.skyboxStars();
+
+ // Create HUD
+ HUD.create("ed3dmap");
+
+ // Add galaxy center
+ Loader.update('Add Sagittarius A*');
+ this.Galaxy.addGalaxyCenter();
+
+ // Load systems
+ Loader.update('Loading JSON file');
+ if(this.jsonPath != null)
+ {
+ Ed3d.loadDatasFromFile();
+ }
+ else if(this.jsonContainer != null)
+ {
+ Ed3d.loadDatasFromContainer();
+ }
+ else if($('.ed3d-item').length > 0)
+ {
+ Ed3d.loadDatasFromAttributes();
+ }
+ else if(this.json != null)
+ {
+ Ed3d.loadDatas(this.json);
+ Ed3d.loadDatasComplete();
+ Ed3d.showScene();
+ }
+ else
+ {
+ Loader.update('No JSON found.');
+ }
+
+ if(!this.startAnim) {
+ Ed3d.grid1XL.hide();
+ this.Galaxy.milkyway2D.visible = false;
+ }
+
+ // Animate
+ animate();
+
+ },
+
+ /**
+ * Init Three.js scene
+ */
+
+ 'loadTextures' : function() {
+
+ //-- Load textures for lensflare
+ var texloader = new THREE.TextureLoader();
+
+ //-- Load textures
+ this.textures.flare_white = texloader.load(Ed3d.basePath + "textures/lensflare/flare2.png");
+ this.textures.flare_yellow = texloader.load(Ed3d.basePath + Ed3d.starSprite);
+ this.textures.flare_center = texloader.load(Ed3d.basePath + "textures/lensflare/flare3.png");
+ this.textures.spiral = texloader.load(Ed3d.basePath + "textures/lensflare/spiral_joe.png");
+ this.textures.permit_zone = texloader.load(Ed3d.basePath + "textures/hydra_invert.jpg");
+
+ //-- Load sprites
+ Ed3d.material.glow_1 = new THREE.SpriteMaterial({
+ map: this.textures.flare_yellow,
+ color: 0xffffff, transparent: false,
+ fog: true
+ });
+ Ed3d.material.glow_2 = new THREE.SpriteMaterial({
+
+ map: Ed3d.textures.flare_white,
+ transparent: true,
+ blending: THREE.AdditiveBlending,
+ depthWrite: false,
+ opacity: 0.5
+ });
+
+ Ed3d.material.spiral = new THREE.SpriteMaterial({
+ map: Ed3d.textures.spiral,
+ transparent: true,
+ blending: THREE.AdditiveBlending,
+ depthWrite: false,
+ opacity: 1
+ });
+
+ Ed3d.material.permit_zone = new THREE.MeshBasicMaterial({
+ map: Ed3d.textures.white,
+ alphaMap: Ed3d.textures.permit_zone,
+ transparent: true,
+ blending: THREE.AdditiveBlending,
+ depthWrite: false,
+ opacity: 0.33
+ });
+
+ },
+
+ 'addCustomMaterial' : function (id, color) {
+
+ var color = new THREE.Color('#'+color);
+ this.colors[id] = color;
+
+ },
+
+
+ /**
+ * Init Three.js scene
+ */
+
+ 'initScene' : function() {
+
+ container = document.getElementById("ed3dmap");
+
+ //Scene
+ scene = new THREE.Scene();
+ scene.visible = false;
+ /*scene.scale.set(10,10,10);*/
+
+ //camera
+ camera = new THREE.PerspectiveCamera(45, container.offsetWidth / container.offsetHeight, 1, 200000);
+ //camera = new THREE.OrthographicCamera( container.offsetWidth / - 2, container.offsetWidth / 2, container.offsetHeight / 2, container.offsetHeight / - 2, - 500, 1000 );
+
+ camera.position.set(0, 500, 500);
+
+ //HemisphereLight
+ light = new THREE.HemisphereLight(0xffffff, 0xcccccc);
+ light.position.set(-0.2, 0.5, 0.8).normalize();
+ scene.add(light);
+
+ //WebGL Renderer
+ renderer = new THREE.WebGLRenderer({
+ antialias: true,
+ alpha: true
+ });
+ renderer.sortObjects = false
+ renderer.setClearColor(0x000000, 1);
+ renderer.setSize(container.offsetWidth, container.offsetHeight);
+ renderer.domElement.style.zIndex = 5;
+ container.appendChild(renderer.domElement);
+
+ //controls
+ controls = new THREE.OrbitControls(camera, container);
+ controls.rotateSpeed = 0.6;
+ controls.zoomSpeed = 2.0;
+ controls.panSpeed = 0.8;
+ controls.maxDistance = 60000;
+ controls.enableZoom=1;controls.enablePan=1;controls.enableDamping=!0;controls.dampingFactor=.3;
+
+
+ // Add Fog
+
+ scene.fog = new THREE.FogExp2(0x0D0D10, 0.000128);
+ renderer.setClearColor(scene.fog.color, 1);
+ Ed3d.fogDensity = scene.fog.density;
+
+ },
+
+ /**
+ * Show the scene when fully loaded
+ */
+
+ 'showScene' : function() {
+
+ Loader.stop();
+ scene.visible = true;
+
+ },
+
+ /**
+ * Load Json file to fill map
+ */
+
+ 'loadDatasFromFile' : function() {
+
+
+ $.getJSON(this.jsonPath, function(data) {
+
+ Ed3d.loadDatas(data);
+
+ }).done(function() {
+
+ Ed3d.loadDatasComplete();
+
+ Ed3d.showScene();
+
+ });
+ },
+
+ 'loadDatasFromContainer' : function() {
+
+ var content = $('#'+this.jsonContainer).html();
+ var json = null;
+
+ try {
+ json = JSON.parse(content);
+ } catch (e) {
+ console.log("Can't load JSon for systems");
+ }
+ if(json != null) Ed3d.loadDatas(json);
+
+ Ed3d.loadDatasComplete();
+
+ Ed3d.showScene();
+
+ },
+
+
+ 'loadDatasFromAttributes' : function() {
+
+ var content = $('#'+this.jsonContainer).html();
+ var json = [];
+ $('.ed3d-item').each(function(e) {
+ var objName = $(this).html();
+ var coords = $(this).data('coords').split(",");
+ if(coords.length == 3)
+ json.push({name:objName,coords:{x:coords[0],y:coords[1],z:coords[2]}});
+ });
+
+ if(json != null) Ed3d.loadDatas(json);
+
+ Ed3d.loadDatasComplete();
+
+ Ed3d.showScene();
+
+ },
+
+
+ 'loadDatas' : function(data) {
+
+ //-- Init Particle system
+ System.initParticleSystem();
+
+ //-- Load cat filters
+ if(data.categories != undefined) HUD.initFilters(data.categories);
+
+ //-- Check if simple or complex json
+ list = (data.systems !== undefined) ? data.systems : data;
+
+ //-- Init Routes
+
+ Loader.update('Routes...');
+ if(data.routes != undefined) {
+ $.each(data.routes, function(key, route) {
+ Route.initRoute(key, route);
+ });
+ }
+
+ //-- Loop into systems
+
+ Loader.update('Systems...');
+ $.each(list, function(key, val) {
+
+ system = System.create(val);
+ if(system != undefined) {
+ if(val.cat != undefined) Ed3d.addObjToCategories(system,val.cat);
+ if(val.cat != undefined) Ed3d.systems.push(system);
+ }
+
+ });
+
+ //-- Routes
+
+ if(data.routes != undefined) {
+
+ $.each(data.routes, function(key, route) {
+ Route.createRoute(key, route);
+ });
+
+ }
+
+ //-- Heatmap
+
+ if(data.heatmap != undefined) {
+ Heatmap.create(data.heatmap);
+ }
+
+ //-- Check start position in JSon
+
+ if(Ed3d.startAnim && data.position != undefined) {
+ Ed3d.playerPos = [data.position.x,data.position.y,data.position.z];
+
+ var camX = (parseInt(data.position.x)-500);
+ var camY = (parseInt(data.position.y)+8500);
+ var camZ = (parseInt(data.position.z)-8500);
+ Ed3d.cameraPos = [camX,camY,camZ];
+
+ Action.moveInitalPosition(4000);
+ }
+
+ },
+
+ 'loadDatasComplete' : function() {
+
+ System.endParticleSystem();
+ HUD.init();
+ this.Action.init();
+
+ },
+
+ /**
+ * Add an object to a category
+ */
+
+ 'addObjToCategories' : function(index, catList) {
+
+ $.each(catList, function(keyArr, idCat) {
+ if(Ed3d.catObjs[idCat] != undefined)
+ Ed3d.catObjs[idCat].push(index);
+ });
+
+ },
+
+ /**
+ * Create a skybox of particle stars
+ */
+
+ 'skyboxStars' : function() {
+
+ var sizeStars = 10000;
+
+ var particles = new THREE.Geometry;
+ for (var p = 0; p < 5; p++) {
+ var particle = new THREE.Vector3(
+ Math.random() * sizeStars - (sizeStars / 2),
+ Math.random() * sizeStars - (sizeStars / 2),
+ Math.random() * sizeStars - (sizeStars / 2)
+ );
+ particles.vertices.push(particle);
+ }
+
+ var particleMaterial = new THREE.PointsMaterial({
+ color: 0xeeeeee,
+ size: 2
+ });
+ this.starfield = new THREE.Points(particles, particleMaterial);
+
+
+ scene.add(this.starfield);
+ },
+
+
+ /**
+ * Calc distance from Sol
+ */
+
+ 'calcDistSol' : function(target) {
+
+ var dx = target.x;
+ var dy = target.y;
+ var dz = target.z;
+
+ return Math.round(Math.sqrt(dx*dx+dy*dy+dz*dz));
+ }
+
+
+}
+
+
+
+//------------------------------------------------------------------------------
+//------------------------------------------------------------------------------
+//------------------------------------------------------------------------------
+//------------------------------------------------------------------------------
+
+function animate(time) {
+
+ //rendererStats.update(renderer);
+
+ if(scene.visible == false) {
+ requestAnimationFrame( animate );
+ return;
+ }
+
+ refreshWithCamPos();
+ //controls.noRotate().set(false);
+ //controls.noPan().set(false);
+ //controls.minPolarAngle = 0;
+ //controls.maxPolarAngle = 0;
+
+ controls.update();
+
+ TWEEN.update(time);
+
+ //-- If 2D top view, lock camera pos
+ if(Ed3d.isTopView) {
+ camera.rotation.set(-Math.PI/2,0,0);
+ camera.position.x = controls.target.x;
+ camera.position.z = controls.target.z;
+ }
+
+
+ renderer.render(scene, camera);
+
+ $('#cx').html(Math.round(controls.target.x));
+ $('#cy').html(Math.round(controls.target.y));
+ $('#cz').html(Math.round(-controls.target.z)); // Reverse z coord
+
+ $('#distsol').html(Ed3d.calcDistSol(controls.target));
+
+ //-- Move starfield with cam
+ Ed3d.starfield.position.set(
+ controls.target.x-(controls.target.x/10)%4000,
+ controls.target.y-(controls.target.y/10)%4000,
+ controls.target.z-(controls.target.z/10)%4000
+ );
+
+ //-- Change selection cursor size depending on camera distance
+
+ var scale = distanceFromTarget(camera)/200;
+
+ this.Action.updateCursorSize(scale);
+
+ HUD.rotateText('system');
+ HUD.rotateText('coords');
+ HUD.rotateText('system_hover');
+
+
+ //-- Zoom on on galaxy effect
+ this.Action.sizeOnScroll(scale);
+
+ this.Galaxy.infosUpdateCallback(scale);
+
+ if(scale>25) {
+
+ enableFarView(scale);
+
+ } else {
+
+ disableFarView(scale);
+
+ }
+
+ this.Action.updatePointClickRadius(scale);
+
+ requestAnimationFrame( animate );
+
+
+}
+
+var isFarView = false;
+
+function enableFarView (scale, withAnim) {
+
+ if(isFarView || this.Galaxy == null) return;
+ if(withAnim == undefined) withAnim = true;
+
+ isFarView = true;
+
+ //-- Scale change animation
+ var scaleFrom = {zoom:25};
+ var scaleTo = {zoom:500};
+ if(withAnim) {
+
+ var obj = this;
+
+ //controls.enabled = false;
+ Ed3d.tween = new TWEEN.Tween(scaleFrom, {override:true}).to(scaleTo, 500)
+ .start()
+ .onUpdate(function () {
+ obj.Galaxy.milkyway[0].material.size = scaleFrom.zoom;
+ obj.Galaxy.milkyway[1].material.size = scaleFrom.zoom*4;
+ });
+
+ } else {
+ this.Galaxy.milkyway[0].material.size = scaleTo;
+ this.Galaxy.milkyway[1].material.size = scaleTo*4;
+ }
+
+ //-- Enable 2D galaxy
+ this.Galaxy.milkyway2D.visible = this.Galaxy.milkyway[0].visible
+ this.Galaxy.infosShow();
+
+
+ //this.Galaxy.obj.scale.set(20,20,20);
+
+ this.Action.updateCursorSize(60);
+
+ Ed3d.grid1H.hide();
+ Ed3d.grid1K.hide();
+ Ed3d.grid1XL.show();
+ Ed3d.starfield.visible = false;
+ scene.fog.density = 0.000009;
+
+}
+
+function disableFarView(scale, withAnim) {
+
+ if(!isFarView) return;
+ if(withAnim == undefined) withAnim = true;
+
+ isFarView = false;
+ var oldScale = parseFloat(1/(25/3));
+
+
+ //-- Scale change animation
+
+ var scaleFrom = {zoom:250};
+ var scaleTo = {zoom:64};
+ if(withAnim) {
+
+ var obj = this;
+
+ //controls.enabled = false;
+ Ed3d.tween = new TWEEN.Tween(scaleFrom, {override:true}).to(scaleTo, 500)
+ .start()
+ .onUpdate(function () {
+ obj.Galaxy.milkyway[0].material.size = scaleFrom.zoom;
+ obj.Galaxy.milkyway[1].material.size = scaleFrom.zoom;
+ });
+
+ } else {
+ this.Galaxy.milkyway[0].material.size = scaleTo;
+ this.Galaxy.milkyway[1].material.size = scaleTo;
+ }
+
+ //-- Disable 2D galaxy
+ this.Galaxy.milkyway2D.visible = false;
+ this.Galaxy.infosHide();
+
+ //-- Show element
+ this.Galaxy.milkyway[0].material.size = 16;
+
+ //--
+ camera.scale.set(1,1,1);
+
+ this.Action.updateCursorSize(1);
+
+ Ed3d.grid1H.show();
+ Ed3d.grid1K.show();
+ Ed3d.grid1XL.hide();
+ Ed3d.starfield.visible = true;
+ scene.fog.density = Ed3d.fogDensity;
+
+}
+
+
+function render() {
+ renderer.render(scene, camera);
+}
+
+function refresh3dMapSize () {
+ if(renderer != undefined) {
+ var width = container.offsetWidth;
+ var height = container.offsetHeight;
+ if(width<100) width = 100;
+ if(height<100) height = 100;
+ renderer.setSize(width, height);
+ camera.aspect = width / height;
+ camera.updateProjectionMatrix();
+ }
+}
+
+
+window.addEventListener('resize', function () {
+ refresh3dMapSize();
+});
+
+
+
+
+
+
+
+
+//--------------------------------------------------------------------------
+// Test perf
+
+
+function distance (v1, v2) {
+ var dx = v1.position.x - v2.position.x;
+ var dy = v1.position.y - v2.position.y;
+ var dz = v1.position.z - v2.position.z;
+
+ return Math.round(Math.sqrt(dx*dx+dy*dy+dz*dz));
+}
+
+function distanceFromTarget (v1) {
+ var dx = v1.position.x - controls.target.x;
+ var dy = v1.position.y - controls.target.y;
+ var dz = v1.position.z - controls.target.z;
+
+ return Math.round(Math.sqrt(dx*dx+dy*dy+dz*dz));
+}
+
+var camSave = {'x':0,'y':0,'z':0};
+
+
+function refreshWithCamPos() {
+
+ var d = new Date();
+ var n = d.getTime();
+
+ //-- Refresh only every 5 sec
+ if(n % 1 != 0) return;
+
+ Ed3d.grid1H.addCoords();
+ Ed3d.grid1K.addCoords();
+
+ //-- Refresh only if the camera moved
+ var p = Ed3d.optDistObj/2;
+ if(
+ camSave.x == Math.round(camera.position.x/p)*p &&
+ camSave.y == Math.round(camera.position.y/p)*p &&
+ camSave.z == Math.round(camera.position.z/p)*p
+ ) return;
+
+ //-- Save new pos
+
+ camSave.x = Math.round(camera.position.x/p)*p;
+ camSave.y = Math.round(camera.position.y/p)*p;
+ camSave.z = Math.round(camera.position.z/p)*p;
+
+}
+
+
+var Loader = {
+
+ /**
+ * Start loader
+ */
+
+ 'start' : function() {
+
+ $('#loader').remove();
+ $('')
+ .attr('id','loader')
+ .html(Loader.svgAnim)
+ .css('color','rgb(200, 110, 37)')
+ .css('font-size','1.5rem')
+ .css('font-family','Helvetica')
+ .css('font-variant','small-caps')
+ .appendTo('#ed3dmap');
+
+
+ clearInterval(this.animCount);
+ this.animCount = setInterval(function () {
+ var animProgress = $('#loader #loadTimer');
+ animProgress.append('.');
+ if(animProgress.html() != undefined && animProgress.html().length > 10) animProgress.html('.');
+ }, 1000);
+
+ },
+
+ /**
+ * Refresh infos for current loading step
+ */
+
+ 'update' : function(info) {
+
+ $('#loader #loadInfos').html(info);
+
+ },
+
+ /**
+ * Stop loader
+ */
+
+ 'stop' : function() {
+
+ $('#loader').remove();
+ clearInterval(this.animCount);
+
+ },
+
+ 'animCount' : null,
+ 'svgAnim' : '.
'
+
+}
\ No newline at end of file
diff --git a/js/ed3dmap.min.js b/js/ed3dmap.min.js
new file mode 100644
index 0000000..98cb5a6
--- /dev/null
+++ b/js/ed3dmap.min.js
@@ -0,0 +1 @@
+var isMinified=true;var camera;var controls;var scene;var light;var renderer;var raycaster;var composer;var container;var routes=[];var lensFlareSel;var Ed3d={container:null,basePath:"./",jsonPath:null,jsonContainer:null,json:null,grid1H:null,grid1K:null,grid1XL:null,tween:null,globalView:true,fogDensity:null,textSel:[],catObjs:[],catObjsRoutes:[],material:{Trd:new THREE.MeshBasicMaterial({color:16777215}),line:new THREE.LineBasicMaterial({color:13421772}),white:new THREE.MeshBasicMaterial({color:16777215}),orange:new THREE.MeshBasicMaterial({color:16751872}),black:new THREE.MeshBasicMaterial({color:65793}),lightblue:new THREE.MeshBasicMaterial({color:950152}),darkblue:new THREE.MeshBasicMaterial({color:1452331}),selected:new THREE.MeshPhongMaterial({color:917503}),grey:new THREE.MeshPhongMaterial({color:8298656}),transparent:new THREE.MeshBasicMaterial({color:0,transparent:true,opacity:0}),glow_1:null,custom:[]},starSprite:"textures/lensflare/star_grey2.png",colors:[],textures:{},systemColor:"#eeeeee",withHudPanel:false,withOptionsPanel:true,hudMultipleSelect:true,systems:[],starfield:null,startAnim:true,effectScaleSystem:[10,800],optDistObj:1500,playerPos:[0,0,0],cameraPos:null,isTopView:false,showGalaxyInfos:false,showNameNear:false,popupDetail:false,Action:null,Galaxy:null,withFullscreenToggle:false,categoryAutoCollapseSize:false,init:function(options){var options=$.extend(Ed3d,options);$("#"+Ed3d.container).append('');Loader.update("Load core files");if(typeof isMinified!=="undefined"){return Ed3d.launchMap()}$.when($.getScript(Ed3d.basePath+"vendor/three-js/OrbitControls.js"),$.getScript(Ed3d.basePath+"vendor/three-js/CSS3DRenderer.js"),$.getScript(Ed3d.basePath+"vendor/three-js/Projector.js"),$.getScript(Ed3d.basePath+"vendor/three-js/FontUtils.js"),$.getScript(Ed3d.basePath+"vendor/three-js/helvetiker_regular.typeface.js"),$.getScript(Ed3d.basePath+"js/components/grid.class.js"),$.getScript(Ed3d.basePath+"js/components/icon.class.js"),$.getScript(Ed3d.basePath+"js/components/hud.class.js"),$.getScript(Ed3d.basePath+"js/components/action.class.js"),$.getScript(Ed3d.basePath+"js/components/route.class.js"),$.getScript(Ed3d.basePath+"js/components/system.class.js"),$.getScript(Ed3d.basePath+"js/components/galaxy.class.js"),$.getScript(Ed3d.basePath+"js/components/heat.class.js"),$.getScript(Ed3d.basePath+"vendor/tween-js/Tween.js"),$.Deferred(function(deferred){$(deferred.resolve)})).done(function(){Loader.update("Done !");Ed3d.launchMap()})},initObjects:function(options){this.Action=Action;this.Galaxy=Galaxy},rebuild:function(options){Loader.start();this.destroy();if(this.jsonPath!=null){Ed3d.loadDatasFromFile()}else{if(this.jsonContainer!=null){Ed3d.loadDatasFromContainer()}}this.Action.moveInitalPosition();Loader.stop()},destroy:function(){Loader.start();System.remove();HUD.removeFilters();Route.remove();Galaxy.remove();Loader.stop()},launchMap:function(){this.initObjects();Loader.update("Textures");Ed3d.loadTextures();Loader.update("Launch scene");Ed3d.initScene();Ed3d.grid1H=$.extend({},Grid.init(100,1121827,0),{});Ed3d.grid1K=$.extend({},Grid.init(1000,2241082,1000),{});Ed3d.grid1XL=$.extend({},Grid.infos(10000,2241082,10000),{});Ed3d.skyboxStars();HUD.create("ed3dmap");Loader.update("Add Sagittarius A*");this.Galaxy.addGalaxyCenter();Loader.update("Loading JSON file");if(this.jsonPath!=null){Ed3d.loadDatasFromFile()}else{if(this.jsonContainer!=null){Ed3d.loadDatasFromContainer()}else{if($(".ed3d-item").length>0){Ed3d.loadDatasFromAttributes()}else{if(this.json!=null){Ed3d.loadDatas(this.json);Ed3d.loadDatasComplete();Ed3d.showScene()}else{Loader.update("No JSON found.")}}}}if(!this.startAnim){Ed3d.grid1XL.hide();this.Galaxy.milkyway2D.visible=false}animate()},loadTextures:function(){var texloader=new THREE.TextureLoader();this.textures.flare_white=texloader.load(Ed3d.basePath+"textures/lensflare/flare2.png");this.textures.flare_yellow=texloader.load(Ed3d.basePath+Ed3d.starSprite);this.textures.flare_center=texloader.load(Ed3d.basePath+"textures/lensflare/flare3.png");Ed3d.material.glow_1=new THREE.SpriteMaterial({map:this.textures.flare_yellow,color:16777215,transparent:false,fog:true});Ed3d.material.glow_2=new THREE.SpriteMaterial({map:Ed3d.textures.flare_white,transparent:true,blending:THREE.AdditiveBlending,depthWrite:false,opacity:0.5})},addCustomMaterial:function(id,color){var color=new THREE.Color("#"+color);this.colors[id]=color},initScene:function(){container=document.getElementById("ed3dmap");scene=new THREE.Scene();scene.visible=false;camera=new THREE.PerspectiveCamera(45,container.offsetWidth/container.offsetHeight,1,200000);camera.position.set(0,500,500);light=new THREE.HemisphereLight(16777215,13421772);light.position.set(-0.2,0.5,0.8).normalize();scene.add(light);renderer=new THREE.WebGLRenderer({antialias:true,alpha:true});renderer.setClearColor(0,1);renderer.setSize(container.offsetWidth,container.offsetHeight);renderer.domElement.style.zIndex=5;container.appendChild(renderer.domElement);controls=new THREE.OrbitControls(camera,container);controls.rotateSpeed=0.6;controls.zoomSpeed=2;controls.panSpeed=0.8;controls.maxDistance=60000;controls.enableZoom=1;controls.enablePan=1;controls.enableDamping=!0;controls.dampingFactor=0.3;scene.fog=new THREE.FogExp2(855312,0.000128);renderer.setClearColor(scene.fog.color,1);Ed3d.fogDensity=scene.fog.density},showScene:function(){Loader.stop();scene.visible=true},loadDatasFromFile:function(){$.getJSON(this.jsonPath,function(data){Ed3d.loadDatas(data)}).done(function(){Ed3d.loadDatasComplete();Ed3d.showScene()})},loadDatasFromContainer:function(){var content=$("#"+this.jsonContainer).html();var json=null;try{json=JSON.parse(content)}catch(e){console.log("Can't load JSon for systems")}if(json!=null){Ed3d.loadDatas(json)}Ed3d.loadDatasComplete();Ed3d.showScene()},loadDatasFromAttributes:function(){var content=$("#"+this.jsonContainer).html();var json=[];$(".ed3d-item").each(function(e){var objName=$(this).html();var coords=$(this).data("coords").split(",");if(coords.length==3){json.push({name:objName,coords:{x:coords[0],y:coords[1],z:coords[2]}})}});if(json!=null){Ed3d.loadDatas(json)}Ed3d.loadDatasComplete();Ed3d.showScene()},loadDatas:function(data){System.initParticleSystem();if(data.categories!=undefined){HUD.initFilters(data.categories)}list=(data.systems!==undefined)?data.systems:data;Loader.update("Routes...");if(data.routes!=undefined){$.each(data.routes,function(key,route){Route.initRoute(key,route)})}Loader.update("Systems...");$.each(list,function(key,val){system=System.create(val);if(system!=undefined){if(val.cat!=undefined){Ed3d.addObjToCategories(system,val.cat)}if(val.cat!=undefined){Ed3d.systems.push(system)}}});if(data.routes!=undefined){$.each(data.routes,function(key,route){Route.createRoute(key,route)})}if(data.heatmap!=undefined){Heatmap.create(data.heatmap)}if(Ed3d.startAnim&&data.position!=undefined){Ed3d.playerPos=[data.position.x,data.position.y,data.position.z];var camX=(parseInt(data.position.x)-500);var camY=(parseInt(data.position.y)+8500);var camZ=(parseInt(data.position.z)-8500);Ed3d.cameraPos=[camX,camY,camZ];Action.moveInitalPosition(4000)}},loadDatasComplete:function(){System.endParticleSystem();HUD.init();this.Action.init()},addObjToCategories:function(index,catList){$.each(catList,function(keyArr,idCat){if(Ed3d.catObjs[idCat]!=undefined){Ed3d.catObjs[idCat].push(index)}})},skyboxStars:function(){var sizeStars=10000;var particles=new THREE.Geometry;for(var p=0;p<5;p++){var particle=new THREE.Vector3(Math.random()*sizeStars-(sizeStars/2),Math.random()*sizeStars-(sizeStars/2),Math.random()*sizeStars-(sizeStars/2));particles.vertices.push(particle)}var particleMaterial=new THREE.PointsMaterial({color:15658734,size:2});this.starfield=new THREE.Points(particles,particleMaterial);scene.add(this.starfield)},calcDistSol:function(target){var dx=target.x;var dy=target.y;var dz=target.z;return Math.round(Math.sqrt(dx*dx+dy*dy+dz*dz))}};function animate(time){if(scene.visible==false){requestAnimationFrame(animate);return}refreshWithCamPos();controls.update();TWEEN.update(time);if(Ed3d.isTopView){camera.rotation.set(-Math.PI/2,0,0);camera.position.x=controls.target.x;camera.position.z=controls.target.z}renderer.render(scene,camera);$("#cx").html(Math.round(controls.target.x));$("#cy").html(Math.round(controls.target.y));$("#cz").html(Math.round(-controls.target.z));$("#distsol").html(Ed3d.calcDistSol(controls.target));Ed3d.starfield.position.set(controls.target.x-(controls.target.x/10)%4000,controls.target.y-(controls.target.y/10)%4000,controls.target.z-(controls.target.z/10)%4000);var scale=distanceFromTarget(camera)/200;this.Action.updateCursorSize(scale);HUD.rotateText("system");HUD.rotateText("coords");HUD.rotateText("system_hover");this.Action.sizeOnScroll(scale);this.Galaxy.infosUpdateCallback(scale);if(scale>25){enableFarView(scale)}else{disableFarView(scale)}this.Action.updatePointClickRadius(scale);requestAnimationFrame(animate)}var isFarView=false;function enableFarView(scale,withAnim){if(isFarView||this.Galaxy==null){return}if(withAnim==undefined){withAnim=true}isFarView=true;var scaleFrom={zoom:25};var scaleTo={zoom:500};if(withAnim){var obj=this;Ed3d.tween=new TWEEN.Tween(scaleFrom,{override:true}).to(scaleTo,500).start().onUpdate(function(){obj.Galaxy.milkyway[0].material.size=scaleFrom.zoom;obj.Galaxy.milkyway[1].material.size=scaleFrom.zoom*4})}else{this.Galaxy.milkyway[0].material.size=scaleTo;this.Galaxy.milkyway[1].material.size=scaleTo*4}this.Galaxy.milkyway2D.visible=true;this.Galaxy.infosShow();this.Action.updateCursorSize(60);Ed3d.grid1H.hide();Ed3d.grid1K.hide();Ed3d.grid1XL.show();Ed3d.starfield.visible=false;scene.fog.density=0.000009}function disableFarView(scale,withAnim){if(!isFarView){return}if(withAnim==undefined){withAnim=true}isFarView=false;var oldScale=parseFloat(1/(25/3));var scaleFrom={zoom:250};var scaleTo={zoom:64};if(withAnim){var obj=this;Ed3d.tween=new TWEEN.Tween(scaleFrom,{override:true}).to(scaleTo,500).start().onUpdate(function(){obj.Galaxy.milkyway[0].material.size=scaleFrom.zoom;obj.Galaxy.milkyway[1].material.size=scaleFrom.zoom})}else{this.Galaxy.milkyway[0].material.size=scaleTo;this.Galaxy.milkyway[1].material.size=scaleTo}this.Galaxy.milkyway2D.visible=false;this.Galaxy.infosHide();this.Galaxy.milkyway[0].material.size=16;camera.scale.set(1,1,1);this.Action.updateCursorSize(1);Ed3d.grid1H.show();Ed3d.grid1K.show();Ed3d.grid1XL.hide();Ed3d.starfield.visible=true;scene.fog.density=Ed3d.fogDensity}function render(){renderer.render(scene,camera)}function refresh3dMapSize(){if(renderer!=undefined){var width=container.offsetWidth;var height=container.offsetHeight;if(width<100){width=100}if(height<100){height=100}renderer.setSize(width,height);camera.aspect=width/height;camera.updateProjectionMatrix()}}window.addEventListener("resize",function(){refresh3dMapSize()});function distance(v1,v2){var dx=v1.position.x-v2.position.x;var dy=v1.position.y-v2.position.y;var dz=v1.position.z-v2.position.z;return Math.round(Math.sqrt(dx*dx+dy*dy+dz*dz))}function distanceFromTarget(v1){var dx=v1.position.x-controls.target.x;var dy=v1.position.y-controls.target.y;var dz=v1.position.z-controls.target.z;return Math.round(Math.sqrt(dx*dx+dy*dy+dz*dz))}var camSave={x:0,y:0,z:0};function refreshWithCamPos(){var d=new Date();var n=d.getTime();if(n%1!=0){return}Ed3d.grid1H.addCoords();Ed3d.grid1K.addCoords();var p=Ed3d.optDistObj/2;if(camSave.x==Math.round(camera.position.x/p)*p&&camSave.y==Math.round(camera.position.y/p)*p&&camSave.z==Math.round(camera.position.z/p)*p){return}camSave.x=Math.round(camera.position.x/p)*p;camSave.y=Math.round(camera.position.y/p)*p;camSave.z=Math.round(camera.position.z/p)*p}var Loader={start:function(){$("#loader").remove();$("").attr("id","loader").html(Loader.svgAnim).css("color","rgb(200, 110, 37)").css("font-size","1.5rem").css("font-family","Helvetica").css("font-variant","small-caps").appendTo("#ed3dmap");clearInterval(this.animCount);this.animCount=setInterval(function(){var animProgress=$("#loader #loadTimer");animProgress.append(".");if(animProgress.html()!=undefined&&animProgress.html().length>10){animProgress.html(".")}},1000)},update:function(info){$("#loader #loadInfos").html(info)},stop:function(){$("#loader").remove();clearInterval(this.animCount)},animCount:null,svgAnim:'.
'};var Action={cursorSel:null,cursorHover:null,cursorScale:1,cursor:{selection:null,hover:null},mouseVector:null,raycaster:null,oldSel:null,objHover:null,mouseUpDownTimer:null,mouseHoverTimer:null,animPosition:null,prevScale:null,pointCastRadius:2,pointsHighlight:[],init:function(){this.mouseVector=new THREE.Vector3();this.raycaster=new THREE.Raycaster();var obj=this;container.addEventListener("mousedown",function(e){obj.onMouseDown(e,obj)},false);container.addEventListener("mouseup",function(e){obj.onMouseUp(e,obj)},false);container.addEventListener("mousemove",function(e){obj.onMouseHover(e,obj)},false);container.addEventListener("mousewheel",this.stopWinScroll,false);container.addEventListener("DOMMouseScroll",this.stopWinScroll,false);if(Ed3d.showNameNear){console.log("Launch EXPERIMENTAL func");window.setInterval(function(){obj.highlightAroundCamera(obj)},1000)}},stopWinScroll:function(event){event.preventDefault();event.stopPropagation()},updatePointClickRadius:function(radius){radius=Math.round(radius);if(radius<2){radius=2}if(this.pointCastRadius==radius){return}this.pointCastRadius=radius},sizeOnScroll:function(scale){if(System.particle==undefined||scale<=0){return}var minScale=Ed3d.effectScaleSystem[0];var maxScale=Ed3d.effectScaleSystem[1];var newScale=scale*20;if(this.prevScale==newScale){return}this.prevScale=newScale;if(newScale>maxScale){newScale=maxScale}if(newScale0){for(var i=0;ilimit){return}var intersection=intersects[i];if(intersection.object.clickable){var indexPoint=intersection.index;var selPoint=intersection.object.geometry.vertices[indexPoint];if(selPoint.visible){var textAdd=selPoint.name;var textId="highlight_"+indexPoint;if(obj.pointsHighlight.indexOf(textId)==-1){HUD.addText(textId,textAdd,0,4,0,1,selPoint,true);obj.pointsHighlight.push(textId)}newSel[textId]=textId}count++}}}$.each(obj.pointsHighlight,function(key,item){if(newSel[item]==undefined){var object=Ed3d.textSel[item];if(object!=undefined){scene.remove(object);Ed3d.textSel.splice(item,1);obj.pointsHighlight.splice(key,1)}}})},onMouseHover:function(e,obj){e.preventDefault();var position=$("#ed3dmap").offset();var scrollPos=$(window).scrollTop();position.top-=scrollPos;obj.mouseVector=new THREE.Vector3(((e.clientX-position.left)/renderer.domElement.width)*2-1,-((e.clientY-position.top)/renderer.domElement.height)*2+1,1);obj.mouseVector.unproject(camera);obj.raycaster=new THREE.Raycaster(camera.position,obj.mouseVector.sub(camera.position).normalize());obj.raycaster.params.Points.threshold=obj.pointCastRadius;var intersects=obj.raycaster.intersectObjects(scene.children);if(intersects.length>0){for(var i=0;i0.2){obj.mouseUpDownTimer=null;return}obj.mouseUpDownTimer=null;var position=$("#ed3dmap").offset();var scrollPos=$(window).scrollTop();position.top-=scrollPos;obj.mouseVector=new THREE.Vector3(((e.clientX-position.left)/renderer.domElement.width)*2-1,-((e.clientY-position.top)/renderer.domElement.height)*2+1,1);obj.mouseVector.unproject(camera);obj.raycaster=new THREE.Raycaster(camera.position,obj.mouseVector.sub(camera.position).normalize());obj.raycaster.params.Points.threshold=obj.pointCastRadius;var intersects=obj.raycaster.intersectObjects(scene.children);if(intersects.length>0){for(var i=0;i"+selPoint.name+"");var isMove=obj.moveToObj(indexPoint,selPoint);var opt=[selPoint.name];var optInfos=(selPoint.infos!=undefined)?selPoint.infos:null;var optUrl=(selPoint.url!=undefined)?selPoint.url:null;$(document).trigger("systemClick",[selPoint.name,optInfos,optUrl]);if(isMove){return}}}if(intersection.object.showCoord){$("#debug").html(Math.round(intersection.point.x)+" , "+Math.round(-intersection.point.z))}}}obj.disableSelection()},moveNextPrev:function(indexPoint,increment){var find=false;while(!find){if(indexPoint<0){indexPoint=System.particleGeo.vertices.length-1}else{if(System.particleGeo.vertices[indexPoint]==undefined){indexPoint=0}}if(System.particleGeo.vertices[indexPoint].visible==true){find=true}else{indexPoint+=increment}}var selPoint=System.particleGeo.vertices[indexPoint];this.moveToObj(indexPoint,selPoint)},disableSelection:function(){if(this.cursor.selection==null){return}this.oldSel=null;this.cursor.selection.visible=false;$("#hud #infos").html("")},moveInitalPositionNoAnim:function(timer){var cam=[Ed3d.playerPos[0],Ed3d.playerPos[1]+500,-Ed3d.playerPos[2]+500];if(Ed3d.cameraPos!=null){cam=[Ed3d.cameraPos[0],Ed3d.cameraPos[1],-Ed3d.cameraPos[2]]}var moveTo={x:cam[0],y:cam[1],z:cam[2],mx:Ed3d.playerPos[0],my:Ed3d.playerPos[1],mz:-Ed3d.playerPos[2]};camera.position.set(moveTo.x,moveTo.y,moveTo.z);controls.target.set(moveTo.mx,moveTo.my,moveTo.mz)},moveInitalPosition:function(timer){if(timer==undefined){timer=800}this.disableSelection();var moveFrom={x:camera.position.x,y:camera.position.y,z:camera.position.z,mx:controls.target.x,my:controls.target.y,mz:controls.target.z};var cam=[Ed3d.playerPos[0],Ed3d.playerPos[1]+500,-Ed3d.playerPos[2]+500];if(Ed3d.cameraPos!=null){cam=[Ed3d.cameraPos[0],Ed3d.cameraPos[1],-Ed3d.cameraPos[2]]}var moveCoords={x:cam[0],y:cam[1],z:cam[2],mx:Ed3d.playerPos[0],my:Ed3d.playerPos[1],mz:-Ed3d.playerPos[2]};controls.enabled=false;if(Ed3d.tween!=null){TWEEN.removeAll()}Ed3d.tween=new TWEEN.Tween(moveFrom,{override:true}).to(moveCoords,timer).start().onUpdate(function(){camera.position.set(moveFrom.x,moveFrom.y,moveFrom.z);controls.target.set(moveFrom.mx,moveFrom.my,moveFrom.mz)}).onComplete(function(){controls.enabled=true;controls.update()})},moveToObj:function(index,obj){if(this.oldSel!==null&&this.oldSel==index){return false}controls.enabled=false;HUD.setInfoPanel(index,obj);if(obj.infos!=undefined){HUD.openHudDetails()}this.oldSel=index;var goX=obj.x;var goY=obj.y;var goZ=obj.z;disableFarView(25,false);this.moveGridTo(goX,goY,goZ);var moveFrom={x:camera.position.x,y:camera.position.y,z:camera.position.z,mx:controls.target.x,my:controls.target.y,mz:controls.target.z};var moveCoords={x:goX,y:goY+15,z:goZ+15,mx:goX,my:goY,mz:goZ};Ed3d.tween=new TWEEN.Tween(moveFrom,{override:true}).to(moveCoords,800).start().onUpdate(function(){camera.position.set(moveFrom.x,moveFrom.y,moveFrom.z);controls.target.set(moveFrom.mx,moveFrom.my,moveFrom.mz)}).onComplete(function(){controls.update()});obj.material=Ed3d.material.selected;this.addCursorOnSelect(goX,goY,goZ);var textAdd=obj.name;var textAddC=Math.round(goX)+", "+Math.round(goY)+", "+Math.round(-goZ);HUD.addText("system",textAdd,8,20,0,6,this.cursor.selection);HUD.addText("coords",textAddC,8,15,0,3,this.cursor.selection);controls.enabled=true;return true},addCursorOnSelect:function(x,y,z){if(this.cursor.selection==null){this.cursor.selection=new THREE.Object3D();var geometryL=new THREE.TorusGeometry(8,0.4,3,20);var selection=new THREE.Mesh(geometryL,Ed3d.material.selected);selection.rotation.x=Math.PI/2;this.cursor.selection.add(selection);var geometryCone=new THREE.CylinderGeometry(0,5,16,4,1,false);var cone=new THREE.Mesh(geometryCone,Ed3d.material.selected);cone.position.set(0,20,0);cone.rotation.x=Math.PI;this.cursor.selection.add(cone);var geometryConeInner=new THREE.CylinderGeometry(0,3.6,16,4,1,false);var coneInner=new THREE.Mesh(geometryConeInner,Ed3d.material.black);coneInner.position.set(0,20.2,0);coneInner.rotation.x=Math.PI;this.cursor.selection.add(coneInner);scene.add(this.cursor.selection)}this.cursor.selection.visible=true;this.cursor.selection.position.set(x,y,z);this.cursor.hover.scale.set(this.cursorScale,this.cursorScale,this.cursorScale)},addCursorOnHover:function(obj){if(this.cursor.hover==null){this.cursor.hover=new THREE.Object3D();var geometryL=new THREE.TorusGeometry(6,0.4,3,20);var selection=new THREE.Mesh(geometryL,Ed3d.material.grey);selection.rotation.x=Math.PI/2;this.cursor.hover.add(selection);scene.add(this.cursor.hover)}this.cursor.hover.position.set(obj.x,obj.y,obj.z);this.cursor.hover.visible=true;this.cursor.hover.scale.set(this.cursorScale,this.cursorScale,this.cursorScale);var textAdd=obj.name;HUD.addText("system_hover",textAdd,0,4,0,3,this.cursor.hover)},updateCursorSize:function(scale){var obj=this;$.each(this.cursor,function(key,cur){if(cur!=null){cur.scale.set(scale,scale,scale)}});this.cursorScale=scale},moveGridTo:function(goX,goY,goZ){var posX=Math.floor(goX/1000)*1000;var posY=Math.floor(goY);var posZ=Math.floor(goZ/1000)*1000;if(!Ed3d.grid1H.fixed){Ed3d.grid1H.obj.position.set(posX,posY,posZ)}if(!Ed3d.grid1K.fixed){Ed3d.grid1K.obj.position.set(posX,posY,posZ)}if(!Ed3d.grid1XL.fixed){Ed3d.grid1XL.obj.position.set(posX,posY,posZ)}}};var Galaxy={obj:null,infos:null,milkyway:[],milkyway2D:null,backActive:true,colors:[],x:25,y:-21,z:25900,Action:null,remove:function(){scene.remove(this.milkyway2D)},addGalaxyCenter:function(){var objVal=new Object;objVal.name="Sagittarius A*";objVal.coords={x:this.y,y:this.y,z:this.z};objVal.cat=[];this.obj=System.create(objVal,true);var sprite=new THREE.Sprite(Ed3d.material.glow_2);sprite.scale.set(50,40,2);this.obj.add(sprite);this.createParticles();this.add2DPlane()},createParticles:function(){var img=new Image();var obj=this;img.onload=function(){obj.getHeightData(img,obj);if(Ed3d.startAnim){camera.position.set(-10000,40000,50000);Action.moveInitalPosition(4000)}else{Action.moveInitalPositionNoAnim()}};img.src=Ed3d.basePath+"textures/heightmap7.jpg";this.showGalaxyInfos()},showGalaxyInfos:function(){if(!Ed3d.showGalaxyInfos){return}this.infos=new THREE.Object3D();var obj=this;$.getJSON(Ed3d.basePath+"data/milkyway-ed.json",function(data){$.each(data.quadrants,function(key,val){obj.addText(key,val.x,-100,val.z,val.rotate)});$.each(data.arms,function(key,val){$.each(val,function(keyCh,valCh){obj.addText(key,valCh.x,0,valCh.z,valCh.rotate,300,true)})});$.each(data.gaps,function(key,val){$.each(val,function(keyCh,valCh){obj.addText(key,valCh.x,0,valCh.z,valCh.rotate,160,true)})});$.each(data.others,function(key,val){$.each(val,function(keyCh,valCh){obj.addText(key,valCh.x,0,valCh.z,valCh.rotate,160,true)})})}).done(function(){scene.add(obj.infos)})},infosShow:function(){if(this.infos==null){this.showGalaxyInfos()}if(this.infos!==null){this.infos.visible=Ed3d.showGalaxyInfos}},infosHide:function(){if(this.infos!==null){this.infos.visible=false}},infosUpdateCallback:function(scale){if(!Ed3d.showGalaxyInfos||this.infos==null){return}scale-=70;var opacity=Math.round(scale/10)/10;if(opacity<0){opacity=0}if(opacity>0.8){opacity=0.8}if(this.infos.previousOpacity==opacity){return}var opacityMiddle=1.1-opacity;if(opacityMiddle<=0.4){opacityMiddle=0.2}for(var i=0;i0.5){i+=8}var all=pix[i]+pix[i+1]+pix[i+2];var avg=Math.round((pix[i]+pix[i+1]+pix[i+2])/3);if(avg>min){var x=scaleImg*((i/4)%img.width);var z=scaleImg*(Math.floor((i/4)/img.height));var density=Math.floor((pix[i]-min)/10);if(density>maxDensity){density=maxDensity}var add=Math.ceil(density/maxDensity*2);for(var y=-density;y=2&&Math.abs(y)-1==0&&Math.random()*1000<200){particlesBig.vertices.push(particle);colorsBig[nbBig]=new THREE.Color("rgb("+r+", "+g+", "+b+")");nbBig++}else{if(density<4||(Math.random()*1000<400-(density*2))){particles.vertices.push(particle);obj.colors[nb]=new THREE.Color("rgb("+r+", "+g+", "+b+")");nb++}}}}}particles.colors=obj.colors;var particleMaterial=new THREE.PointsMaterial({map:Ed3d.textures.flare_yellow,transparent:true,size:64,vertexColors:THREE.VertexColors,blending:THREE.AdditiveBlending,depthTest:true,depthWrite:false});var points=new THREE.Points(particles,particleMaterial);points.sortParticles=true;particles.center();obj.milkyway[0]=points;obj.milkyway[0].scale.set(20,20,20);obj.obj.add(points);particlesBig.colors=colorsBig;var particleMaterialBig=new THREE.PointsMaterial({map:Ed3d.textures.flare_yellow,transparent:true,vertexColors:THREE.VertexColors,size:16,blending:THREE.AdditiveBlending,depthTest:true,depthWrite:false});var pointsBig=new THREE.Points(particlesBig,particleMaterialBig);pointsBig.sortParticles=true;particlesBig.center();obj.milkyway[1]=pointsBig;obj.milkyway[1].scale.set(20,20,20);obj.obj.add(pointsBig)}};var Grid={obj:null,size:null,textShapes:null,textGeo:null,coordTxt:null,minDistView:null,visible:true,fixed:false,init:function(size,color,minDistView){this.size=size;this.obj=new THREE.GridHelper(1000000,size);this.obj.setColors(color,color);this.obj.minDistView=minDistView;scene.add(this.obj);this.obj.customUpdateCallback=this.addCoords;return this},infos:function(step,color,minDistView){var size=50000;if(step==undefined){step=10000}this.fixed=true;var geometry=new THREE.Geometry();var material=new THREE.LineBasicMaterial({color:5592405,transparent:true,opacity:0.2,blending:THREE.AdditiveBlending,depthWrite:false});for(var i=-size;i<=size;i+=step){geometry.vertices.push(new THREE.Vector3(-size,0,i));geometry.vertices.push(new THREE.Vector3(size,0,i));geometry.vertices.push(new THREE.Vector3(i,0,-size));geometry.vertices.push(new THREE.Vector3(i,0,size))}this.obj=new THREE.LineSegments(geometry,material);this.obj.position.set(0,0,-20000);var quadrant=new THREE.Geometry();var material=new THREE.LineBasicMaterial({color:8947848,transparent:true,opacity:0.5,blending:THREE.AdditiveBlending,depthWrite:false});quadrant.vertices.push(new THREE.Vector3(-size,0,20000));quadrant.vertices.push(new THREE.Vector3(size,0,20000));quadrant.vertices.push(new THREE.Vector3(0,0,-size));quadrant.vertices.push(new THREE.Vector3(0,0,size));var quadrantL=new THREE.LineSegments(quadrant,material);this.obj.add(quadrantL);scene.add(this.obj);return this},addCoords:function(){var textShow="0 : 0 : 0";var options={font:"helvetiker",weight:"normal",style:"normal",size:this.size/20,curveSegments:10};if(this.coordGrid!=null){if(Math.abs(camera.position.y-this.obj.position.y)>this.size*10||Math.abs(camera.position.y-this.obj.position.y)heatColor.length-1){sizeColor=heatColor.length-1}colors[count]=heatColor[sizeColor][0]/255;colors[count+1]=heatColor[sizeColor][1]/255;colors[count+2]=heatColor[sizeColor][2]/255;count+=3;var height=Math.abs(prop.y[1]-prop.y[0])*2;if(height<100){height=100}var geometry=new THREE.BoxBufferGeometry(170,height,170);var material=new THREE.MeshLambertMaterial({color:"rgb("+heatColor[sizeColor][0]+", "+heatColor[sizeColor][1]+", "+heatColor[sizeColor][2]+")"});var cube=new THREE.Mesh(geometry,material);cube.position.set(prop.x,height/2,-prop.z);scene.add(cube)});geometry.addAttribute("position",new THREE.BufferAttribute(positions,3));geometry.addAttribute("color",new THREE.BufferAttribute(colors,3));var particles=new THREE.Points(geometry,particleMaterial)}};var HUD={container:null,init:function(){Loader.update("Init HUD");this.initHudAction();this.initControls()},create:function(container){this.container=container;if(!$("#"+this.container+" #controls").length&&Ed3d.withOptionsPanel==true){$("#"+this.container).append(' ');this.createSubOptions();if(Ed3d.withFullscreenToggle){$("").attr("id","tog-fullscreen").html("Fullscreen").click(function(){$("#"+container).toggleClass("map-fullscreen");refresh3dMapSize()}).prependTo("#controls")}}if(!Ed3d.withHudPanel){return}$("#"+this.container).append('');$("#hud").append('
');var addClass=(Ed3d.popupDetail?'class="popup-detail"':"");$("#"+this.container).append('")},createSubOptions:function(){$("").addClass("sub-opt active").html("Toggle Milky Way").click(function(){var state=Galaxy.milkyway[0].visible;Galaxy.milkyway[0].visible=!state;Galaxy.milkyway[1].visible=!state;Galaxy.milkyway2D.visible=!state;$(this).toggleClass("active")}).appendTo("#options");$("").addClass("sub-opt active").html("Toggle grid").click(function(){Ed3d.grid1H.toggleGrid();Ed3d.grid1K.toggleGrid();Ed3d.grid1XL.toggleGrid();$(this).toggleClass("active")}).appendTo("#options")},initControls:function(){$("#controls a").click(function(e){if($(this).hasClass("view")){$("#controls a.view").removeClass("selected");$(this).addClass("selected")}var view=$(this).data("view");switch(view){case"top":Ed3d.isTopView=true;var moveFrom={x:camera.position.x,y:camera.position.y,z:camera.position.z};var moveCoords={x:controls.target.x,y:controls.target.y+500,z:controls.target.z};HUD.moveCamera(moveFrom,moveCoords);break;case"3d":Ed3d.isTopView=false;var moveFrom={x:camera.position.x,y:camera.position.y,z:camera.position.z};var moveCoords={x:controls.target.x-100,y:controls.target.y+500,z:controls.target.z+500};HUD.moveCamera(moveFrom,moveCoords);break;case"infos":if(!Ed3d.showGalaxyInfos){Ed3d.showGalaxyInfos=true;Galaxy.infosShow()}else{Ed3d.showGalaxyInfos=false;Galaxy.infosHide()}$(this).toggleClass("selected");break;case"options":$("#options").toggle();break}})},moveCamera:function(from,to){Ed3d.tween=new TWEEN.Tween(from,{override:true}).to(to,800).start().onUpdate(function(){camera.position.set(from.x,from.y,from.z)}).onComplete(function(){controls.update()})},initHudAction:function(){$("canvas").hover(function(){controls.enabled=true},function(){controls.enabled=false});$("#hud").hover(function(){controls.enabled=false},function(){controls.enabled=true});$("#systemDetails").hide();$(".map_filter").each(function(e){var idCat=$(this).data("filter");var count=Ed3d.catObjs[idCat].length;if(count>1){$(this).append(" ("+count+")")}});$(".map_filter").click(function(e){e.preventDefault();var idCat=$(this).data("filter");var active=$(this).data("active");active=(Math.abs(active-1));if(!Ed3d.hudMultipleSelect){$(".map_filter").addClass("disabled");$(System.particleGeo.vertices).each(function(index,point){point.visible=0;point.filtered=0;System.particleGeo.colors[index]=new THREE.Color("#111111");active=1});if(Ed3d.catObjsRoutes.length>0){$(Ed3d.catObjsRoutes).each(function(indexCat,listGrpRoutes){if(listGrpRoutes!=undefined){$(listGrpRoutes).each(function(key,indexRoute){scene.getObjectByName(indexRoute).visible=false;if(scene.getObjectByName(indexRoute+"-first")!=undefined){scene.getObjectByName(indexRoute+"-first").visible=false}if(scene.getObjectByName(indexRoute+"-last")!=undefined){scene.getObjectByName(indexRoute+"-last").visible=false}})}})}}var center=null;var nbPoint=0;var pointFar=null;if(Ed3d.catObjsRoutes.length>0){$(Ed3d.catObjsRoutes[idCat]).each(function(key,indexRoute){var isVisible=scene.getObjectByName(indexRoute).visible;if(isVisible==undefined){isVisible=true}isVisible=(isVisible?false:true);scene.getObjectByName(indexRoute).visible=isVisible;if(scene.getObjectByName(indexRoute+"-first")!=undefined){scene.getObjectByName(indexRoute+"-first").visible=isVisible}if(scene.getObjectByName(indexRoute+"-last")!=undefined){scene.getObjectByName(indexRoute+"-last").visible=isVisible}})}$(Ed3d.catObjs[idCat]).each(function(key,indexPoint){obj=System.particleGeo.vertices[indexPoint];System.particleGeo.colors[indexPoint]=(active==1)?obj.color:new THREE.Color("#111111");obj.visible=(active==1);obj.filtered=(active==1);System.particleGeo.colorsNeedUpdate=true;if(center==null){center=new THREE.Vector3(obj.x,obj.y,obj.z);pointFar=new THREE.Vector3(obj.x,obj.y,obj.z)}else{center.set((center.x+obj.x),(center.y+obj.y),(center.z+obj.z));if((Math.abs(pointFar.x)-Math.abs(obj.x))+(Math.abs(pointFar.y)-Math.abs(obj.y))+(Math.abs(pointFar.z)-Math.abs(obj.z))<0){pointFar.set(obj.x,obj.y,obj.z)}}nbPoint++});if(nbPoint==0){return}center.set(Math.round(center.x/nbPoint),Math.round(center.y/nbPoint),-Math.round(center.z/nbPoint));$(this).data("active",active);$(this).toggleClass("disabled");if(Action.oldSel!=null&&!Action.oldSel.visible){Action.disableSelection()}var distance=pointFar.distanceTo(center)+200;Ed3d.playerPos=[center.x,center.y,center.z];Ed3d.cameraPos=[center.x+(Math.floor((Math.random()*100)+1)-50),center.y+distance,center.z-distance];Action.moveInitalPosition()});$(".map_link").click(function(e){e.preventDefault();var elId=$(this).data("route");Action.moveToObj(routes[elId])});$(".map_link span").click(function(e){e.preventDefault();var elId=$(this).parent().data("route");routes[elId].visible=!routes[elId].visible})},initFilters:function(categories){Loader.update("HUD Filter...");var grpNb=1;$.each(categories,function(typeFilter,values){if(typeof values==="object"){var groupId="group_"+grpNb;var nbFilters=values.length;var count=0;var visible=true;$("#filters").append(""+typeFilter+"
");$("#filters").append('');$.each(values,function(key,val){visible=true;if(Ed3d.categoryAutoCollapseSize!==false){count++;if(count>Ed3d.categoryAutoCollapseSize){visible=false}}HUD.addFilter(groupId,key,val,visible);Ed3d.catObjs[key]=[]});grpNb++;if(visible==false){$("#"+groupId).append('+ See more').click(function(){HUD.expandFilters(groupId)})}}})},expandFilters:function(groupId){$("#"+groupId).addClass("open");$("#hud").addClass("enlarge")},removeFilters:function(){$("#hud #filters").html("")},addFilter:function(groupId,idCat,val,visible){var back="#fff";var addClass="";if(val.color!=undefined){Ed3d.addCustomMaterial(idCat,val.color);back="#"+val.color}if(!visible){addClass+=" hidden"}$("#"+groupId).append(' '+val.name+"")},openHudDetails:function(){$("#hud").hide();$("#systemDetails").show().hover(function(){controls.enabled=false},function(){controls.enabled=true})},closeHudDetails:function(){$("#hud").show();$("#systemDetails").hide()},setRoute:function(idRoute,nameR){$("#routes").append(' '+nameR+"")},setInfoPanel:function(index,point){$("#systemDetails").html(""+point.name+'
'+point.x+""+point.y+""+(-point.z)+'
'+(point.infos!=undefined?""+point.infos+"
":"")+'');$("",{html:"<"}).click(function(){Action.moveNextPrev(index-1,-1)}).appendTo("#nav");$("",{html:"X"}).click(function(){HUD.closeHudDetails()}).appendTo("#nav");$("",{html:">"}).click(function(){Action.moveNextPrev(index+1,1)}).appendTo("#nav")},addText:function(id,textShow,x,y,z,size,addToObj,isPoint){if(addToObj==undefined){addToObj=scene}if(isPoint==undefined){isPoint=false}var textShapes=THREE.FontUtils.generateShapes(textShow,{font:"helvetiker",weight:"normal",style:"normal",size:size,curveSegments:100});var textGeo=new THREE.ShapeGeometry(textShapes);if(Ed3d.textSel[id]==undefined){var textMesh=new THREE.Mesh(textGeo,new THREE.MeshBasicMaterial({color:16777215}))}else{var textMesh=Ed3d.textSel[id]}textMesh.geometry=textGeo;textMesh.geometry.needsUpdate=true;if(isPoint){textMesh.position.set(addToObj.x,addToObj.y,addToObj.z);textMesh.name=id;scene.add(textMesh)}else{textMesh.position.set(x,y,z);addToObj.add(textMesh)}Ed3d.textSel[id]=textMesh},rotateText:function(id){if(Ed3d.textSel[id]!=undefined){if(Ed3d.isTopView){Ed3d.textSel[id].rotation.set(-Math.PI/2,0,0)}else{Ed3d.textSel[id].rotation.x=0;Ed3d.textSel[id].rotation.y=camera.rotation.y;Ed3d.textSel[id].rotation.z=0}}}};var Ico={cog:''};var Route={active:false,systems:[],list:[],initRoute:function(idRoute,route){Route.active=true;$.each(route.points,function(key,val){Route.systems[val.s]=false;if(val.coords!=undefined){val.name=val.s;System.create(val)}})},remove:function(){$(Ed3d.catObjsRoutes).each(function(indexCat,listGrpRoutes){if(listGrpRoutes!=undefined){$(listGrpRoutes).each(function(key,indexRoute){scene.remove(scene.getObjectByName(indexRoute));if(scene.getObjectByName(indexRoute+"-first")!=undefined){scene.remove(scene.getObjectByName(indexRoute+"-first"))}if(scene.getObjectByName(indexRoute+"-last")!=undefined){scene.remove(scene.getObjectByName(indexRoute+"-last"))}})}})},createRoute:function(idRoute,route){var geometryL=new THREE.Geometry();var nameR="";var first=null;var last=null;var color=Ed3d.material.orange;var colorLine=Ed3d.material.line;var hideLast=(route.hideLast!==undefined&&route.hideLast);if(route.cat!==undefined&&route.cat[0]!=undefined&&Ed3d.colors[route.cat[0]]!=undefined){color=new THREE.MeshBasicMaterial({color:Ed3d.colors[route.cat[0]]});colorLine=new THREE.MeshBasicMaterial({color:Ed3d.colors[route.cat[0]]})}else{color=Ed3d.material.orange}$.each(route.points,function(key2,val){if(Route.systems[val.s]!==false){var c=Route.systems[val.s];if(first==null){first=[c[0],c[1],c[2]]}last=[c[0],c[1],c[2]];geometryL.vertices.push(new THREE.Vector3(c[0],c[1],c[2]))}else{console.log("Missing point: "+val.s)}});routes[idRoute]=new THREE.Line(geometryL,colorLine);if(first!==null){this.addCircle("route-"+idRoute+"-first",first,color,7)}if(!hideLast&&last!==null){this.addCircle("route-"+idRoute+"-last",last,color,3)}routes[idRoute].name="route-"+idRoute;scene.add(routes[idRoute]);if(route.cat!==undefined){$.each(route.cat,function(keyArr,idCat){if(Ed3d.catObjsRoutes[idCat]==undefined){Ed3d.catObjsRoutes[idCat]=[]}Ed3d.catObjsRoutes[idCat].push(routes[idRoute].name)})}},addCircle:function(id,point,material,size){var cursor=new THREE.Object3D;var geometryL=new THREE.TorusGeometry(size,0.4,3,15);var selection=new THREE.Mesh(geometryL,material);selection.rotation.x=Math.PI/2;cursor.add(selection);cursor.position.set(point[0],point[1],point[2]);cursor.scale.set(15,15,15);cursor.name=id;scene.add(cursor);Action.cursor[cursor.id]=cursor},addPoint:function(x,y,z,name){var cursor=new THREE.Object3D;var geometryL=new THREE.TorusGeometry(12,0.4,3,30);var selection=new THREE.Mesh(geometryL,Ed3d.material.selected);selection.rotation.x=Math.PI/2;cursor.add(selection);var geometryCone=new THREE.CylinderGeometry(0,5,16,4,1,false);var cone=new THREE.Mesh(geometryCone,Ed3d.material.selected);cone.position.set(0,20,0);cone.rotation.x=Math.PI;cursor.add(cone);var geometryConeInner=new THREE.CylinderGeometry(0,3.6,16,4,1,false);var coneInner=new THREE.Mesh(geometryConeInner,Ed3d.material.black);coneInner.position.set(0,20.2,0);coneInner.rotation.x=Math.PI;cursor.add(coneInner);cursor.position.set(x,y,z);cursor.scale.set(30,30,30);scene.add(cursor)},newRoute:function(name){var routes=localStorage.getItem("routes");if(routes==undefined){var routes=[]}var myRoute={title:"Test route",points:[]};routes.push(myRoute);routes.push(myRoute);routes.push(myRoute);localStorage.setItem("routes",JSON.stringify(routes))},addPointToRoute:function(x,y,z,system,label){idRoute=1;console.log("add point");var routes=JSON.parse(localStorage.getItem("routes"));if(routes[idRoute]==undefined){return false}var point={coords:{x:x,y:y,z:z}};if(system==undefined){system=Math.floor(Date.now()/1000)}if(system!=undefined){point.s=system}if(label!=undefined){point.label=label}routes[idRoute].points.push(point);localStorage.setItem("routes",JSON.stringify(routes));this.drawRoute(1)},drawRoute:function(idRoute){var route=JSON.parse(localStorage.getItem("routes"))[idRoute];if(route==undefined){return false}this.initRoute(0,route);this.createRoute(0,route)}};var System={particle:null,particleGeo:null,particleColor:[],particleInfos:[],count:0,scaleSize:64,create:function(val,withSolid){if(withSolid==undefined){withSolid=false}if(val.coords==undefined){return false}var x=parseInt(val.coords.x);var y=parseInt(val.coords.y);var z=-parseInt(val.coords.z);var colors=[];if(this.particleGeo!==null){var idSys=x+"_"+y+"_"+z;if(val.infos!=undefined&&this.particleInfos[idSys]){var indexParticle=this.particleInfos[idSys];this.particleGeo.vertices[indexParticle].infos+=val.infos;if(val.cat!=undefined){Ed3d.addObjToCategories(indexParticle,val.cat)}return}var particle=new THREE.Vector3(x,y,z);if(val.cat!=undefined&&val.cat[0]!=undefined&&Ed3d.colors[val.cat[0]]!=undefined){this.particleColor[this.count]=Ed3d.colors[val.cat[0]]}else{this.particleColor[this.count]=new THREE.Color(Ed3d.systemColor)}if(val.cat!=undefined){Ed3d.addObjToCategories(this.count,val.cat);particle.color=this.particleColor[this.count]}particle.clickable=true;particle.visible=true;particle.name=val.name;if(val.infos!=undefined){particle.infos=val.infos;this.particleInfos[idSys]=this.count}if(val.url!=undefined){particle.url=val.url}this.particleGeo.vertices.push(particle);this.count++}if(Route.active==true){if(Route.systems[val.name]!=undefined){Route.systems[val.name]=[x,y,z]}}if(withSolid){var mat=Ed3d.material.glow_1;var sprite=new THREE.Sprite(mat);sprite.position.set(x,y,z);sprite.scale.set(50,50,1);scene.add(sprite);var geometry=new THREE.SphereGeometry(2,10,10);var sphere=new THREE.Mesh(geometry,Ed3d.material.white);sphere.position.set(x,y,z);sphere.name=val.name;sphere.clickable=true;sphere.idsprite=sprite.id;scene.add(sphere);return sphere}},initParticleSystem:function(){this.particleGeo=new THREE.Geometry},endParticleSystem:function(){if(this.particleGeo==null){return}this.particleGeo.colors=this.particleColor;var particleMaterial=new THREE.PointsMaterial({map:Ed3d.textures.flare_yellow,vertexColors:THREE.VertexColors,size:this.scaleSize,fog:false,blending:THREE.AdditiveBlending,transparent:true,depthTest:true,depthWrite:false});this.particle=new THREE.Points(this.particleGeo,particleMaterial);this.particle.sortParticles=true;this.particle.clickable=true;scene.add(this.particle)},remove:function(){this.particleColor=[];this.particleGeo=null;this.count=0;scene.remove(this.particle)},loadSpectral:function(val){}};THREE.CSS3DObject=function(element){THREE.Object3D.call(this);this.element=element;this.element.style.position="absolute";this.addEventListener("removed",function(event){if(this.element.parentNode!==null){this.element.parentNode.removeChild(this.element)}})};THREE.CSS3DObject.prototype=Object.create(THREE.Object3D.prototype);THREE.CSS3DObject.prototype.constructor=THREE.CSS3DObject;THREE.CSS3DSprite=function(element){THREE.CSS3DObject.call(this,element)};THREE.CSS3DSprite.prototype=Object.create(THREE.CSS3DObject.prototype);THREE.CSS3DSprite.prototype.constructor=THREE.CSS3DSprite;THREE.CSS3DRenderer=function(){console.log("THREE.CSS3DRenderer",THREE.REVISION);var _width,_height;var _widthHalf,_heightHalf;var matrix=new THREE.Matrix4();var cache={camera:{fov:0,style:""},objects:{}};var domElement=document.createElement("div");domElement.style.overflow="hidden";domElement.style.WebkitTransformStyle="preserve-3d";domElement.style.MozTransformStyle="preserve-3d";domElement.style.oTransformStyle="preserve-3d";domElement.style.transformStyle="preserve-3d";this.domElement=domElement;var cameraElement=document.createElement("div");cameraElement.style.WebkitTransformStyle="preserve-3d";cameraElement.style.MozTransformStyle="preserve-3d";cameraElement.style.oTransformStyle="preserve-3d";cameraElement.style.transformStyle="preserve-3d";domElement.appendChild(cameraElement);this.setClearColor=function(){};this.getSize=function(){return{width:_width,height:_height}};this.setSize=function(width,height){_width=width;_height=height;_widthHalf=_width/2;_heightHalf=_height/2;domElement.style.width=width+"px";domElement.style.height=height+"px";cameraElement.style.width=width+"px";cameraElement.style.height=height+"px"};var epsilon=function(value){return Math.abs(value)":{x_min:18.0625,x_max:774,ha:792,o:"m 774 376 l 18 40 l 18 149 l 631 421 l 18 692 l 18 799 l 774 465 l 774 376 "},v:{x_min:0,x_max:675.15625,ha:761,o:"m 675 738 l 404 0 l 272 0 l 0 738 l 133 737 l 340 147 l 541 737 l 675 738 "},"τ":{x_min:0.28125,x_max:644.5,ha:703,o:"m 644 628 l 382 628 l 382 179 q 388 120 382 137 q 436 91 401 91 q 474 94 447 91 q 504 97 501 97 l 504 0 q 454 -9 482 -5 q 401 -14 426 -14 q 278 67 308 -14 q 260 233 260 118 l 260 628 l 0 628 l 0 739 l 644 739 l 644 628 "},"ξ":{x_min:0,x_max:624.9375,ha:699,o:"m 624 -37 q 608 -153 624 -96 q 563 -278 593 -211 l 454 -278 q 491 -183 486 -200 q 511 -83 511 -126 q 484 -23 511 -44 q 370 1 452 1 q 323 0 354 1 q 283 -1 293 -1 q 84 76 169 -1 q 0 266 0 154 q 56 431 0 358 q 197 538 108 498 q 94 613 134 562 q 54 730 54 665 q 77 823 54 780 q 143 901 101 867 l 27 901 l 27 1012 l 576 1012 l 576 901 l 380 901 q 244 863 303 901 q 178 745 178 820 q 312 600 178 636 q 532 582 380 582 l 532 479 q 276 455 361 479 q 118 281 118 410 q 165 173 118 217 q 274 120 208 133 q 494 101 384 110 q 624 -37 624 76 "},"&":{x_min:-3,x_max:894.25,ha:992,o:"m 894 0 l 725 0 l 624 123 q 471 0 553 40 q 306 -41 390 -41 q 168 -7 231 -41 q 62 92 105 26 q 14 187 31 139 q -3 276 -3 235 q 55 433 -3 358 q 248 581 114 508 q 170 689 196 640 q 137 817 137 751 q 214 985 137 922 q 384 1041 284 1041 q 548 988 483 1041 q 622 824 622 928 q 563 666 622 739 q 431 556 516 608 l 621 326 q 649 407 639 361 q 663 493 653 426 l 781 493 q 703 229 781 352 l 894 0 m 504 818 q 468 908 504 877 q 384 940 433 940 q 293 907 331 940 q 255 818 255 875 q 289 714 255 767 q 363 628 313 678 q 477 729 446 682 q 504 818 504 771 m 556 209 l 314 499 q 179 395 223 449 q 135 283 135 341 q 146 222 135 253 q 183 158 158 192 q 333 80 241 80 q 556 209 448 80 "},"Λ":{x_min:0,x_max:862.5,ha:942,o:"m 862 0 l 719 0 l 426 847 l 143 0 l 0 0 l 356 1013 l 501 1013 l 862 0 "},I:{x_min:41,x_max:180,ha:293,o:"m 180 0 l 41 0 l 41 1013 l 180 1013 l 180 0 "},G:{x_min:0,x_max:921,ha:1011,o:"m 921 0 l 832 0 l 801 136 q 655 15 741 58 q 470 -28 568 -28 q 126 133 259 -28 q 0 499 0 284 q 125 881 0 731 q 486 1043 259 1043 q 763 957 647 1043 q 905 709 890 864 l 772 709 q 668 866 747 807 q 486 926 589 926 q 228 795 322 926 q 142 507 142 677 q 228 224 142 342 q 483 94 323 94 q 712 195 625 94 q 796 435 796 291 l 477 435 l 477 549 l 921 549 l 921 0 "},"ΰ":{x_min:0,x_max:617,ha:725,o:"m 524 800 l 414 800 l 414 925 l 524 925 l 524 800 m 183 800 l 73 800 l 73 925 l 183 925 l 183 800 m 617 352 q 540 93 617 199 q 308 -24 455 -24 q 76 93 161 -24 q 0 352 0 199 l 0 738 l 126 738 l 126 354 q 169 185 126 257 q 312 98 220 98 q 451 185 402 98 q 492 354 492 257 l 492 738 l 617 738 l 617 352 m 489 1040 l 300 819 l 216 819 l 351 1040 l 489 1040 "},"`":{x_min:0,x_max:138.890625,ha:236,o:"m 138 699 l 0 699 l 0 861 q 36 974 0 929 q 138 1041 72 1020 l 138 977 q 82 931 95 969 q 69 839 69 893 l 138 839 l 138 699 "},"·":{x_min:0,x_max:142,ha:239,o:"m 142 585 l 0 585 l 0 738 l 142 738 l 142 585 "},"Υ":{x_min:0.328125,x_max:819.515625,ha:889,o:"m 819 1013 l 482 416 l 482 0 l 342 0 l 342 416 l 0 1013 l 140 1013 l 411 533 l 679 1013 l 819 1013 "},r:{x_min:0,x_max:355.5625,ha:432,o:"m 355 621 l 343 621 q 179 569 236 621 q 122 411 122 518 l 122 0 l 0 0 l 0 737 l 117 737 l 117 604 q 204 719 146 686 q 355 753 262 753 l 355 621 "},x:{x_min:0,x_max:675,ha:764,o:"m 675 0 l 525 0 l 331 286 l 144 0 l 0 0 l 256 379 l 12 738 l 157 737 l 336 473 l 516 738 l 661 738 l 412 380 l 675 0 "},"μ":{x_min:0,x_max:696.609375,ha:747,o:"m 696 -4 q 628 -14 657 -14 q 498 97 513 -14 q 422 8 470 41 q 313 -24 374 -24 q 207 3 258 -24 q 120 80 157 31 l 120 -278 l 0 -278 l 0 738 l 124 738 l 124 343 q 165 172 124 246 q 308 82 216 82 q 451 177 402 82 q 492 358 492 254 l 492 738 l 616 738 l 616 214 q 623 136 616 160 q 673 92 636 92 q 696 95 684 92 l 696 -4 "},h:{x_min:0,x_max:615,ha:724,o:"m 615 472 l 615 0 l 490 0 l 490 454 q 456 590 490 535 q 338 654 416 654 q 186 588 251 654 q 122 436 122 522 l 122 0 l 0 0 l 0 1013 l 122 1013 l 122 633 q 218 727 149 694 q 362 760 287 760 q 552 676 484 760 q 615 472 615 600 "},".":{x_min:0,x_max:142,ha:239,o:"m 142 0 l 0 0 l 0 151 l 142 151 l 142 0 "},"φ":{x_min:-2,x_max:878,ha:974,o:"m 496 -279 l 378 -279 l 378 -17 q 101 88 204 -17 q -2 367 -2 194 q 68 626 -2 510 q 283 758 151 758 l 283 646 q 167 537 209 626 q 133 373 133 462 q 192 177 133 254 q 378 93 259 93 l 378 758 q 445 764 426 763 q 476 765 464 765 q 765 659 653 765 q 878 377 878 553 q 771 96 878 209 q 496 -17 665 -17 l 496 -279 m 496 93 l 514 93 q 687 183 623 93 q 746 380 746 265 q 691 569 746 491 q 522 658 629 658 l 496 656 l 496 93 "},";":{x_min:0,x_max:142,ha:239,o:"m 142 585 l 0 585 l 0 738 l 142 738 l 142 585 m 142 -12 q 105 -132 142 -82 q 0 -206 68 -182 l 0 -138 q 58 -82 43 -123 q 68 0 68 -56 l 0 0 l 0 151 l 142 151 l 142 -12 "},f:{x_min:0,x_max:378,ha:472,o:"m 378 638 l 246 638 l 246 0 l 121 0 l 121 638 l 0 638 l 0 738 l 121 738 q 137 935 121 887 q 290 1028 171 1028 q 320 1027 305 1028 q 378 1021 334 1026 l 378 908 q 323 918 346 918 q 257 870 273 918 q 246 780 246 840 l 246 738 l 378 738 l 378 638 "},"“":{x_min:1,x_max:348.21875,ha:454,o:"m 140 670 l 1 670 l 1 830 q 37 943 1 897 q 140 1011 74 990 l 140 947 q 82 900 97 940 q 68 810 68 861 l 140 810 l 140 670 m 348 670 l 209 670 l 209 830 q 245 943 209 897 q 348 1011 282 990 l 348 947 q 290 900 305 940 q 276 810 276 861 l 348 810 l 348 670 "},A:{x_min:0.03125,x_max:906.953125,ha:1008,o:"m 906 0 l 756 0 l 648 303 l 251 303 l 142 0 l 0 0 l 376 1013 l 529 1013 l 906 0 m 610 421 l 452 867 l 293 421 l 610 421 "},"6":{x_min:53,x_max:739,ha:792,o:"m 739 312 q 633 62 739 162 q 400 -31 534 -31 q 162 78 257 -31 q 53 439 53 206 q 178 859 53 712 q 441 986 284 986 q 643 912 559 986 q 732 713 732 833 l 601 713 q 544 830 594 786 q 426 875 494 875 q 268 793 331 875 q 193 517 193 697 q 301 597 240 570 q 427 624 362 624 q 643 540 552 624 q 739 312 739 451 m 603 298 q 540 461 603 400 q 404 516 484 516 q 268 461 323 516 q 207 300 207 401 q 269 137 207 198 q 405 83 325 83 q 541 137 486 83 q 603 298 603 197 "},"‘":{x_min:1,x_max:139.890625,ha:236,o:"m 139 670 l 1 670 l 1 830 q 37 943 1 897 q 139 1011 74 990 l 139 947 q 82 900 97 940 q 68 810 68 861 l 139 810 l 139 670 "},"ϊ":{x_min:-70,x_max:283,ha:361,o:"m 283 800 l 173 800 l 173 925 l 283 925 l 283 800 m 40 800 l -70 800 l -70 925 l 40 925 l 40 800 m 283 3 q 232 -10 257 -5 q 181 -15 206 -15 q 84 26 118 -15 q 41 200 41 79 l 41 737 l 166 737 l 167 215 q 171 141 167 157 q 225 101 182 101 q 247 103 238 101 q 283 112 256 104 l 283 3 "},"π":{x_min:-0.21875,x_max:773.21875,ha:857,o:"m 773 -7 l 707 -11 q 575 40 607 -11 q 552 174 552 77 l 552 226 l 552 626 l 222 626 l 222 0 l 97 0 l 97 626 l 0 626 l 0 737 l 773 737 l 773 626 l 676 626 l 676 171 q 695 103 676 117 q 773 90 714 90 l 773 -7 "},"ά":{x_min:0,x_max:765.5625,ha:809,o:"m 765 -4 q 698 -14 726 -14 q 564 97 586 -14 q 466 7 525 40 q 337 -26 407 -26 q 88 98 186 -26 q 0 369 0 212 q 88 637 0 525 q 337 760 184 760 q 465 727 407 760 q 563 637 524 695 l 563 738 l 685 738 l 685 222 q 693 141 685 168 q 748 94 708 94 q 765 95 760 94 l 765 -4 m 584 371 q 531 562 584 485 q 360 653 470 653 q 192 566 254 653 q 135 379 135 489 q 186 181 135 261 q 358 84 247 84 q 528 176 465 84 q 584 371 584 260 m 604 1040 l 415 819 l 332 819 l 466 1040 l 604 1040 "},O:{x_min:0,x_max:958,ha:1057,o:"m 485 1041 q 834 882 702 1041 q 958 512 958 734 q 834 136 958 287 q 481 -26 702 -26 q 126 130 261 -26 q 0 504 0 279 q 127 880 0 728 q 485 1041 263 1041 m 480 98 q 731 225 638 98 q 815 504 815 340 q 733 783 815 669 q 480 912 640 912 q 226 784 321 912 q 142 504 142 670 q 226 224 142 339 q 480 98 319 98 "},n:{x_min:0,x_max:615,ha:724,o:"m 615 463 l 615 0 l 490 0 l 490 454 q 453 592 490 537 q 331 656 410 656 q 178 585 240 656 q 117 421 117 514 l 117 0 l 0 0 l 0 738 l 117 738 l 117 630 q 218 728 150 693 q 359 764 286 764 q 552 675 484 764 q 615 463 615 593 "},"3":{x_min:54,x_max:737,ha:792,o:"m 737 284 q 635 55 737 141 q 399 -25 541 -25 q 156 52 248 -25 q 54 308 54 140 l 185 308 q 245 147 185 202 q 395 96 302 96 q 539 140 484 96 q 602 280 602 190 q 510 429 602 390 q 324 454 451 454 l 324 565 q 487 584 441 565 q 565 719 565 617 q 515 835 565 791 q 395 879 466 879 q 255 824 307 879 q 203 661 203 769 l 78 661 q 166 909 78 822 q 387 992 250 992 q 603 921 513 992 q 701 723 701 844 q 669 607 701 656 q 578 524 637 558 q 696 434 655 499 q 737 284 737 369 "},"9":{x_min:53,x_max:739,ha:792,o:"m 739 524 q 619 94 739 241 q 362 -32 516 -32 q 150 47 242 -32 q 59 244 59 126 l 191 244 q 246 129 191 176 q 373 82 301 82 q 526 161 466 82 q 597 440 597 255 q 363 334 501 334 q 130 432 216 334 q 53 650 53 521 q 134 880 53 786 q 383 986 226 986 q 659 841 566 986 q 739 524 739 719 m 388 449 q 535 514 480 449 q 585 658 585 573 q 535 805 585 744 q 388 873 480 873 q 242 809 294 873 q 191 658 191 745 q 239 514 191 572 q 388 449 292 449 "},l:{x_min:41,x_max:166,ha:279,o:"m 166 0 l 41 0 l 41 1013 l 166 1013 l 166 0 "},"¤":{x_min:40.09375,x_max:728.796875,ha:825,o:"m 728 304 l 649 224 l 512 363 q 383 331 458 331 q 256 363 310 331 l 119 224 l 40 304 l 177 441 q 150 553 150 493 q 184 673 150 621 l 40 818 l 119 898 l 267 749 q 321 766 291 759 q 384 773 351 773 q 447 766 417 773 q 501 749 477 759 l 649 898 l 728 818 l 585 675 q 612 618 604 648 q 621 553 621 587 q 591 441 621 491 l 728 304 m 384 682 q 280 643 318 682 q 243 551 243 604 q 279 461 243 499 q 383 423 316 423 q 487 461 449 423 q 525 553 525 500 q 490 641 525 605 q 384 682 451 682 "},"κ":{x_min:0,x_max:632.328125,ha:679,o:"m 632 0 l 482 0 l 225 384 l 124 288 l 124 0 l 0 0 l 0 738 l 124 738 l 124 446 l 433 738 l 596 738 l 312 466 l 632 0 "},"4":{x_min:48,x_max:742.453125,ha:792,o:"m 742 243 l 602 243 l 602 0 l 476 0 l 476 243 l 48 243 l 48 368 l 476 958 l 602 958 l 602 354 l 742 354 l 742 243 m 476 354 l 476 792 l 162 354 l 476 354 "},p:{x_min:0,x_max:685,ha:786,o:"m 685 364 q 598 96 685 205 q 350 -23 504 -23 q 121 89 205 -23 l 121 -278 l 0 -278 l 0 738 l 121 738 l 121 633 q 220 726 159 691 q 351 761 280 761 q 598 636 504 761 q 685 364 685 522 m 557 371 q 501 560 557 481 q 330 651 437 651 q 162 559 223 651 q 108 366 108 479 q 162 177 108 254 q 333 87 224 87 q 502 178 441 87 q 557 371 557 258 "},"‡":{x_min:0,x_max:777,ha:835,o:"m 458 238 l 458 0 l 319 0 l 319 238 l 0 238 l 0 360 l 319 360 l 319 681 l 0 683 l 0 804 l 319 804 l 319 1015 l 458 1013 l 458 804 l 777 804 l 777 683 l 458 683 l 458 360 l 777 360 l 777 238 l 458 238 "},"ψ":{x_min:0,x_max:808,ha:907,o:"m 465 -278 l 341 -278 l 341 -15 q 87 102 180 -15 q 0 378 0 210 l 0 739 l 133 739 l 133 379 q 182 195 133 275 q 341 98 242 98 l 341 922 l 465 922 l 465 98 q 623 195 563 98 q 675 382 675 278 l 675 742 l 808 742 l 808 381 q 720 104 808 213 q 466 -13 627 -13 l 465 -278 "},"η":{x_min:0.78125,x_max:697,ha:810,o:"m 697 -278 l 572 -278 l 572 454 q 540 587 572 536 q 425 650 501 650 q 271 579 337 650 q 206 420 206 509 l 206 0 l 81 0 l 81 489 q 73 588 81 562 q 0 644 56 644 l 0 741 q 68 755 38 755 q 158 720 124 755 q 200 630 193 686 q 297 726 234 692 q 434 761 359 761 q 620 692 544 761 q 697 516 697 624 l 697 -278 "}},cssFontWeight:"normal",ascender:1189,underlinePosition:-100,cssFontStyle:"normal",boundingBox:{yMin:-334,xMin:-111,yMax:1189,xMax:1672},resolution:1000,original_font_information:{postscript_name:"Helvetiker-Regular",version_string:"Version 1.00 2004 initial release",vendor_url:"http://www.magenta.gr/",full_font_name:"Helvetiker",font_family_name:"Helvetiker",copyright:"Copyright (c) Μagenta ltd, 2004",description:"",trademark:"",designer:"",designer_url:"",unique_font_identifier:"Μagenta ltd:Helvetiker:22-10-104",license_url:"http://www.ellak.gr/fonts/MgOpen/license.html",license_description:'Copyright (c) 2004 by MAGENTA Ltd. All Rights Reserved.\r\n\r\nPermission is hereby granted, free of charge, to any person obtaining a copy of the fonts accompanying this license ("Fonts") and associated documentation files (the "Font Software"), to reproduce and distribute the Font Software, including without limitation the rights to use, copy, merge, publish, distribute, and/or sell copies of the Font Software, and to permit persons to whom the Font Software is furnished to do so, subject to the following conditions: \r\n\r\nThe above copyright and this permission notice shall be included in all copies of one or more of the Font Software typefaces.\r\n\r\nThe Font Software may be modified, altered, or added to, and in particular the designs of glyphs or characters in the Fonts may be modified and additional glyphs or characters may be added to the Fonts, only if the fonts are renamed to names not containing the word "MgOpen", or if the modifications are accepted for inclusion in the Font Software itself by the each appointed Administrator.\r\n\r\nThis License becomes null and void to the extent applicable to Fonts or Font Software that has been modified and is distributed under the "MgOpen" name.\r\n\r\nThe Font Software may be sold as part of a larger software package but no copy of one or more of the Font Software typefaces may be sold by itself. \r\n\r\nTHE FONT SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO ANY WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT OF COPYRIGHT, PATENT, TRADEMARK, OR OTHER RIGHT. IN NO EVENT SHALL MAGENTA OR PERSONS OR BODIES IN CHARGE OF ADMINISTRATION AND MAINTENANCE OF THE FONT SOFTWARE BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, INCLUDING ANY GENERAL, SPECIAL, INDIRECT, INCIDENTAL, OR CONSEQUENTIAL DAMAGES, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF THE USE OR INABILITY TO USE THE FONT SOFTWARE OR FROM OTHER DEALINGS IN THE FONT SOFTWARE.',manufacturer_name:"Μagenta ltd",font_sub_family_name:"Regular"},descender:-334,familyName:"Helvetiker",lineHeight:1522,underlineThickness:50})}THREE.OrbitControls=function(object,domElement){this.object=object;this.domElement=(domElement!==undefined)?domElement:document;this.enabled=true;this.target=new THREE.Vector3();this.minDistance=0;this.maxDistance=Infinity;this.minZoom=0;this.maxZoom=Infinity;this.minPolarAngle=0;this.maxPolarAngle=Math.PI;this.minAzimuthAngle=-Infinity;this.maxAzimuthAngle=Infinity;this.enableDamping=false;this.dampingFactor=0.25;this.enableZoom=true;this.zoomSpeed=1;this.enableRotate=true;this.rotateSpeed=1;this.enablePan=true;this.keyPanSpeed=7;this.autoRotate=false;this.autoRotateSpeed=2;this.enableKeys=true;this.keys={LEFT:37,UP:38,RIGHT:39,BOTTOM:40};this.mouseButtons={ORBIT:THREE.MOUSE.LEFT,ZOOM:THREE.MOUSE.MIDDLE,PAN:THREE.MOUSE.RIGHT};this.target0=this.target.clone();this.position0=this.object.position.clone();this.zoom0=this.object.zoom;this.getPolarAngle=function(){return spherical.phi};this.getAzimuthalAngle=function(){return spherical.theta};this.reset=function(){scope.target.copy(scope.target0);scope.object.position.copy(scope.position0);scope.object.zoom=scope.zoom0;scope.object.updateProjectionMatrix();scope.dispatchEvent(changeEvent);scope.update();state=STATE.NONE};this.update=function(){var offset=new THREE.Vector3();var quat=new THREE.Quaternion().setFromUnitVectors(object.up,new THREE.Vector3(0,1,0));var quatInverse=quat.clone().inverse();var lastPosition=new THREE.Vector3();var lastQuaternion=new THREE.Quaternion();return function(){var position=scope.object.position;offset.copy(position).sub(scope.target);offset.applyQuaternion(quat);spherical.setFromVector3(offset);if(scope.autoRotate&&state===STATE.NONE){rotateLeft(getAutoRotationAngle())}spherical.theta+=sphericalDelta.theta;spherical.phi+=sphericalDelta.phi;spherical.theta=Math.max(scope.minAzimuthAngle,Math.min(scope.maxAzimuthAngle,spherical.theta));spherical.phi=Math.max(scope.minPolarAngle,Math.min(scope.maxPolarAngle,spherical.phi));spherical.makeSafe();spherical.radius*=scale;spherical.radius=Math.max(scope.minDistance,Math.min(scope.maxDistance,spherical.radius));scope.target.add(panOffset);offset.setFromSpherical(spherical);offset.applyQuaternion(quatInverse);position.copy(scope.target).add(offset);scope.object.lookAt(scope.target);if(scope.enableDamping===true){sphericalDelta.theta*=(1-scope.dampingFactor);sphericalDelta.phi*=(1-scope.dampingFactor)}else{sphericalDelta.set(0,0,0)}scale=1;panOffset.set(0,0,0);if(zoomChanged||lastPosition.distanceToSquared(scope.object.position)>EPS||8*(1-lastQuaternion.dot(scope.object.quaternion))>EPS){scope.dispatchEvent(changeEvent);lastPosition.copy(scope.object.position);lastQuaternion.copy(scope.object.quaternion);zoomChanged=false;return true}return false}}();this.dispose=function(){scope.domElement.removeEventListener("contextmenu",onContextMenu,false);scope.domElement.removeEventListener("mousedown",onMouseDown,false);scope.domElement.removeEventListener("mousewheel",onMouseWheel,false);scope.domElement.removeEventListener("MozMousePixelScroll",onMouseWheel,false);scope.domElement.removeEventListener("touchstart",onTouchStart,false);scope.domElement.removeEventListener("touchend",onTouchEnd,false);scope.domElement.removeEventListener("touchmove",onTouchMove,false);document.removeEventListener("mousemove",onMouseMove,false);document.removeEventListener("mouseup",onMouseUp,false);document.removeEventListener("mouseout",onMouseUp,false);window.removeEventListener("keydown",onKeyDown,false)};var scope=this;var changeEvent={type:"change"};var startEvent={type:"start"};var endEvent={type:"end"};var STATE={NONE:-1,ROTATE:0,DOLLY:1,PAN:2,TOUCH_ROTATE:3,TOUCH_DOLLY:4,TOUCH_PAN:5};var state=STATE.NONE;var EPS=0.000001;var spherical=new THREE.Spherical();var sphericalDelta=new THREE.Spherical();var scale=1;var panOffset=new THREE.Vector3();var zoomChanged=false;var rotateStart=new THREE.Vector2();var rotateEnd=new THREE.Vector2();var rotateDelta=new THREE.Vector2();var panStart=new THREE.Vector2();var panEnd=new THREE.Vector2();var panDelta=new THREE.Vector2();var dollyStart=new THREE.Vector2();var dollyEnd=new THREE.Vector2();var dollyDelta=new THREE.Vector2();function getAutoRotationAngle(){return 2*Math.PI/60/60*scope.autoRotateSpeed}function getZoomScale(){return Math.pow(0.95,scope.zoomSpeed)}function rotateLeft(angle){sphericalDelta.theta-=angle}function rotateUp(angle){sphericalDelta.phi-=angle}var panLeft=function(){var v=new THREE.Vector3();return function panLeft(distance,objectMatrix){v.setFromMatrixColumn(objectMatrix,0);v.multiplyScalar(-distance);panOffset.add(v)}}();var panUp=function(){var v=new THREE.Vector3();return function panUp(distance,objectMatrix){v.setFromMatrixColumn(objectMatrix,1);v.multiplyScalar(distance);panOffset.add(v)}}();var pan=function(){var offset=new THREE.Vector3();return function(deltaX,deltaY){var element=scope.domElement===document?scope.domElement.body:scope.domElement;if(scope.object instanceof THREE.PerspectiveCamera){var position=scope.object.position;offset.copy(position).sub(scope.target);var targetDistance=offset.length();targetDistance*=Math.tan((scope.object.fov/2)*Math.PI/180);panLeft(2*deltaX*targetDistance/element.clientHeight,scope.object.matrix);panUp(2*deltaY*targetDistance/element.clientHeight,scope.object.matrix)}else{if(scope.object instanceof THREE.OrthographicCamera){panLeft(deltaX*(scope.object.right-scope.object.left)/scope.object.zoom/element.clientWidth,scope.object.matrix);panUp(deltaY*(scope.object.top-scope.object.bottom)/scope.object.zoom/element.clientHeight,scope.object.matrix)}else{console.warn("WARNING: OrbitControls.js encountered an unknown camera type - pan disabled.");scope.enablePan=false}}}}();function dollyIn(dollyScale){if(scope.object instanceof THREE.PerspectiveCamera){scale/=dollyScale}else{if(scope.object instanceof THREE.OrthographicCamera){scope.object.zoom=Math.max(scope.minZoom,Math.min(scope.maxZoom,scope.object.zoom*dollyScale));scope.object.updateProjectionMatrix();zoomChanged=true}else{console.warn("WARNING: OrbitControls.js encountered an unknown camera type - dolly/zoom disabled.");scope.enableZoom=false}}}function dollyOut(dollyScale){if(scope.object instanceof THREE.PerspectiveCamera){scale*=dollyScale}else{if(scope.object instanceof THREE.OrthographicCamera){scope.object.zoom=Math.max(scope.minZoom,Math.min(scope.maxZoom,scope.object.zoom/dollyScale));scope.object.updateProjectionMatrix();zoomChanged=true}else{console.warn("WARNING: OrbitControls.js encountered an unknown camera type - dolly/zoom disabled.");scope.enableZoom=false}}}function handleMouseDownRotate(event){rotateStart.set(event.clientX,event.clientY)}function handleMouseDownDolly(event){dollyStart.set(event.clientX,event.clientY)}function handleMouseDownPan(event){panStart.set(event.clientX,event.clientY)}function handleMouseMoveRotate(event){rotateEnd.set(event.clientX,event.clientY);rotateDelta.subVectors(rotateEnd,rotateStart);var element=scope.domElement===document?scope.domElement.body:scope.domElement;rotateLeft(2*Math.PI*rotateDelta.x/element.clientWidth*scope.rotateSpeed);rotateUp(2*Math.PI*rotateDelta.y/element.clientHeight*scope.rotateSpeed);rotateStart.copy(rotateEnd);scope.update()}function handleMouseMoveDolly(event){dollyEnd.set(event.clientX,event.clientY);dollyDelta.subVectors(dollyEnd,dollyStart);if(dollyDelta.y>0){dollyIn(getZoomScale())}else{if(dollyDelta.y<0){dollyOut(getZoomScale())}}dollyStart.copy(dollyEnd);scope.update()}function handleMouseMovePan(event){panEnd.set(event.clientX,event.clientY);panDelta.subVectors(panEnd,panStart);pan(panDelta.x,panDelta.y);panStart.copy(panEnd);scope.update()}function handleMouseUp(event){}function handleMouseWheel(event){var delta=0;if(event.wheelDelta!==undefined){delta=event.wheelDelta}else{if(event.detail!==undefined){delta=-event.detail}}if(delta>0){dollyOut(getZoomScale())}else{if(delta<0){dollyIn(getZoomScale())}}scope.update()}function handleKeyDown(event){switch(event.keyCode){case scope.keys.UP:pan(0,scope.keyPanSpeed);scope.update();break;case scope.keys.BOTTOM:pan(0,-scope.keyPanSpeed);scope.update();break;case scope.keys.LEFT:pan(scope.keyPanSpeed,0);scope.update();break;case scope.keys.RIGHT:pan(-scope.keyPanSpeed,0);scope.update();break}}function handleTouchStartRotate(event){rotateStart.set(event.touches[0].pageX,event.touches[0].pageY)}function handleTouchStartDolly(event){var dx=event.touches[0].pageX-event.touches[1].pageX;var dy=event.touches[0].pageY-event.touches[1].pageY;var distance=Math.sqrt(dx*dx+dy*dy);dollyStart.set(0,distance)}function handleTouchStartPan(event){panStart.set(event.touches[0].pageX,event.touches[0].pageY)}function handleTouchMoveRotate(event){rotateEnd.set(event.touches[0].pageX,event.touches[0].pageY);rotateDelta.subVectors(rotateEnd,rotateStart);var element=scope.domElement===document?scope.domElement.body:scope.domElement;rotateLeft(2*Math.PI*rotateDelta.x/element.clientWidth*scope.rotateSpeed);rotateUp(2*Math.PI*rotateDelta.y/element.clientHeight*scope.rotateSpeed);rotateStart.copy(rotateEnd);scope.update()}function handleTouchMoveDolly(event){var dx=event.touches[0].pageX-event.touches[1].pageX;var dy=event.touches[0].pageY-event.touches[1].pageY;var distance=Math.sqrt(dx*dx+dy*dy);dollyEnd.set(0,distance);dollyDelta.subVectors(dollyEnd,dollyStart);if(dollyDelta.y>0){dollyOut(getZoomScale())}else{if(dollyDelta.y<0){dollyIn(getZoomScale())}}dollyStart.copy(dollyEnd);scope.update()}function handleTouchMovePan(event){panEnd.set(event.touches[0].pageX,event.touches[0].pageY);panDelta.subVectors(panEnd,panStart);pan(panDelta.x,panDelta.y);panStart.copy(panEnd);scope.update()}function handleTouchEnd(event){}function onMouseDown(event){if(scope.enabled===false){return}event.preventDefault();if(event.button===scope.mouseButtons.ORBIT){if(scope.enableRotate===false){return}handleMouseDownRotate(event);state=STATE.ROTATE}else{if(event.button===scope.mouseButtons.ZOOM){if(scope.enableZoom===false){return}handleMouseDownDolly(event);state=STATE.DOLLY}else{if(event.button===scope.mouseButtons.PAN){if(scope.enablePan===false){return}handleMouseDownPan(event);state=STATE.PAN}}}if(state!==STATE.NONE){document.addEventListener("mousemove",onMouseMove,false);document.addEventListener("mouseup",onMouseUp,false);document.addEventListener("mouseout",onMouseUp,false);scope.dispatchEvent(startEvent)}}function onMouseMove(event){if(scope.enabled===false){return}event.preventDefault();if(state===STATE.ROTATE){if(scope.enableRotate===false){return}handleMouseMoveRotate(event)}else{if(state===STATE.DOLLY){if(scope.enableZoom===false){return}handleMouseMoveDolly(event)}else{if(state===STATE.PAN){if(scope.enablePan===false){return}handleMouseMovePan(event)}}}}function onMouseUp(event){if(scope.enabled===false){return}handleMouseUp(event);document.removeEventListener("mousemove",onMouseMove,false);document.removeEventListener("mouseup",onMouseUp,false);document.removeEventListener("mouseout",onMouseUp,false);scope.dispatchEvent(endEvent);state=STATE.NONE}function onMouseWheel(event){if(scope.enabled===false||scope.enableZoom===false||(state!==STATE.NONE&&state!==STATE.ROTATE)){return}event.preventDefault();event.stopPropagation();handleMouseWheel(event);scope.dispatchEvent(startEvent);scope.dispatchEvent(endEvent)}function onKeyDown(event){if(scope.enabled===false||scope.enableKeys===false||scope.enablePan===false){return}handleKeyDown(event)}function onTouchStart(event){if(scope.enabled===false){return}switch(event.touches.length){case 1:if(scope.enableRotate===false){return}handleTouchStartRotate(event);state=STATE.TOUCH_ROTATE;break;case 2:if(scope.enableZoom===false){return}handleTouchStartDolly(event);state=STATE.TOUCH_DOLLY;break;case 3:if(scope.enablePan===false){return}handleTouchStartPan(event);state=STATE.TOUCH_PAN;break;default:state=STATE.NONE}if(state!==STATE.NONE){scope.dispatchEvent(startEvent)}}function onTouchMove(event){if(scope.enabled===false){return}event.preventDefault();event.stopPropagation();switch(event.touches.length){case 1:if(scope.enableRotate===false){return}if(state!==STATE.TOUCH_ROTATE){return}handleTouchMoveRotate(event);break;case 2:if(scope.enableZoom===false){return}if(state!==STATE.TOUCH_DOLLY){return}handleTouchMoveDolly(event);break;case 3:if(scope.enablePan===false){return}if(state!==STATE.TOUCH_PAN){return}handleTouchMovePan(event);break;default:state=STATE.NONE}}function onTouchEnd(event){if(scope.enabled===false){return}handleTouchEnd(event);scope.dispatchEvent(endEvent);state=STATE.NONE}function onContextMenu(event){event.preventDefault()}scope.domElement.addEventListener("contextmenu",onContextMenu,false);scope.domElement.addEventListener("mousedown",onMouseDown,false);scope.domElement.addEventListener("mousewheel",onMouseWheel,false);scope.domElement.addEventListener("MozMousePixelScroll",onMouseWheel,false);scope.domElement.addEventListener("touchstart",onTouchStart,false);scope.domElement.addEventListener("touchend",onTouchEnd,false);scope.domElement.addEventListener("touchmove",onTouchMove,false);window.addEventListener("keydown",onKeyDown,false);this.update()};THREE.OrbitControls.prototype=Object.create(THREE.EventDispatcher.prototype);THREE.OrbitControls.prototype.constructor=THREE.OrbitControls;Object.defineProperties(THREE.OrbitControls.prototype,{center:{get:function(){console.warn("THREE.OrbitControls: .center has been renamed to .target");return this.target}},noZoom:{get:function(){console.warn("THREE.OrbitControls: .noZoom has been deprecated. Use .enableZoom instead.");return !this.enableZoom},set:function(value){console.warn("THREE.OrbitControls: .noZoom has been deprecated. Use .enableZoom instead.");this.enableZoom=!value}},noRotate:{get:function(){console.warn("THREE.OrbitControls: .noRotate has been deprecated. Use .enableRotate instead.");return !this.enableRotate},set:function(value){console.warn("THREE.OrbitControls: .noRotate has been deprecated. Use .enableRotate instead.");this.enableRotate=!value}},noPan:{get:function(){console.warn("THREE.OrbitControls: .noPan has been deprecated. Use .enablePan instead.");return !this.enablePan},set:function(value){console.warn("THREE.OrbitControls: .noPan has been deprecated. Use .enablePan instead.");this.enablePan=!value}},noKeys:{get:function(){console.warn("THREE.OrbitControls: .noKeys has been deprecated. Use .enableKeys instead.");return !this.enableKeys},set:function(value){console.warn("THREE.OrbitControls: .noKeys has been deprecated. Use .enableKeys instead.");this.enableKeys=!value}},staticMoving:{get:function(){console.warn("THREE.OrbitControls: .staticMoving has been deprecated. Use .enableDamping instead.");return !this.enableDamping},set:function(value){console.warn("THREE.OrbitControls: .staticMoving has been deprecated. Use .enableDamping instead.");this.enableDamping=!value}},dynamicDampingFactor:{get:function(){console.warn("THREE.OrbitControls: .dynamicDampingFactor has been renamed. Use .dampingFactor instead.");return this.dampingFactor},set:function(value){console.warn("THREE.OrbitControls: .dynamicDampingFactor has been renamed. Use .dampingFactor instead.");this.dampingFactor=value}}});THREE.RenderableObject=function(){this.id=0;this.object=null;this.z=0;this.renderOrder=0};THREE.RenderableFace=function(){this.id=0;this.v1=new THREE.RenderableVertex();this.v2=new THREE.RenderableVertex();this.v3=new THREE.RenderableVertex();this.normalModel=new THREE.Vector3();this.vertexNormalsModel=[new THREE.Vector3(),new THREE.Vector3(),new THREE.Vector3()];this.vertexNormalsLength=0;this.color=new THREE.Color();this.material=null;this.uvs=[new THREE.Vector2(),new THREE.Vector2(),new THREE.Vector2()];this.z=0;this.renderOrder=0};THREE.RenderableVertex=function(){this.position=new THREE.Vector3();this.positionWorld=new THREE.Vector3();this.positionScreen=new THREE.Vector4();this.visible=true};THREE.RenderableVertex.prototype.copy=function(vertex){this.positionWorld.copy(vertex.positionWorld);this.positionScreen.copy(vertex.positionScreen)};THREE.RenderableLine=function(){this.id=0;this.v1=new THREE.RenderableVertex();this.v2=new THREE.RenderableVertex();this.vertexColors=[new THREE.Color(),new THREE.Color()];this.material=null;this.z=0;this.renderOrder=0};THREE.RenderableSprite=function(){this.id=0;this.object=null;this.x=0;this.y=0;this.z=0;this.rotation=0;this.scale=new THREE.Vector2();this.material=null;this.renderOrder=0};THREE.Projector=function(){var _object,_objectCount,_objectPool=[],_objectPoolLength=0,_vertex,_vertexCount,_vertexPool=[],_vertexPoolLength=0,_face,_faceCount,_facePool=[],_facePoolLength=0,_line,_lineCount,_linePool=[],_linePoolLength=0,_sprite,_spriteCount,_spritePool=[],_spritePoolLength=0,_renderData={objects:[],lights:[],elements:[]},_vector3=new THREE.Vector3(),_vector4=new THREE.Vector4(),_clipBox=new THREE.Box3(new THREE.Vector3(-1,-1,-1),new THREE.Vector3(1,1,1)),_boundingBox=new THREE.Box3(),_points3=new Array(3),_points4=new Array(4),_viewMatrix=new THREE.Matrix4(),_viewProjectionMatrix=new THREE.Matrix4(),_modelMatrix,_modelViewProjectionMatrix=new THREE.Matrix4(),_normalMatrix=new THREE.Matrix3(),_frustum=new THREE.Frustum(),_clippedVertex1PositionScreen=new THREE.Vector4(),_clippedVertex2PositionScreen=new THREE.Vector4();this.projectVector=function(vector,camera){console.warn("THREE.Projector: .projectVector() is now vector.project().");vector.project(camera)};this.unprojectVector=function(vector,camera){console.warn("THREE.Projector: .unprojectVector() is now vector.unproject().");vector.unproject(camera)};this.pickingRay=function(vector,camera){console.error("THREE.Projector: .pickingRay() is now raycaster.setFromCamera().")};var RenderList=function(){var normals=[];var uvs=[];var object=null;var material=null;var normalMatrix=new THREE.Matrix3();function setObject(value){object=value;material=object.material;normalMatrix.getNormalMatrix(object.matrixWorld);normals.length=0;uvs.length=0}function projectVertex(vertex){var position=vertex.position;var positionWorld=vertex.positionWorld;var positionScreen=vertex.positionScreen;positionWorld.copy(position).applyMatrix4(_modelMatrix);positionScreen.copy(positionWorld).applyMatrix4(_viewProjectionMatrix);var invW=1/positionScreen.w;positionScreen.x*=invW;positionScreen.y*=invW;positionScreen.z*=invW;vertex.visible=positionScreen.x>=-1&&positionScreen.x<=1&&positionScreen.y>=-1&&positionScreen.y<=1&&positionScreen.z>=-1&&positionScreen.z<=1}function pushVertex(x,y,z){_vertex=getNextVertexInPool();_vertex.position.set(x,y,z);projectVertex(_vertex)}function pushNormal(x,y,z){normals.push(x,y,z)}function pushUv(x,y){uvs.push(x,y)}function checkTriangleVisibility(v1,v2,v3){if(v1.visible===true||v2.visible===true||v3.visible===true){return true}_points3[0]=v1.positionScreen;_points3[1]=v2.positionScreen;_points3[2]=v3.positionScreen;return _clipBox.intersectsBox(_boundingBox.setFromPoints(_points3))}function checkBackfaceCulling(v1,v2,v3){return((v3.positionScreen.x-v1.positionScreen.x)*(v2.positionScreen.y-v1.positionScreen.y)-(v3.positionScreen.y-v1.positionScreen.y)*(v2.positionScreen.x-v1.positionScreen.x))<0}function pushLine(a,b){var v1=_vertexPool[a];var v2=_vertexPool[b];_line=getNextLineInPool();_line.id=object.id;_line.v1.copy(v1);_line.v2.copy(v2);_line.z=(v1.positionScreen.z+v2.positionScreen.z)/2;_line.renderOrder=object.renderOrder;_line.material=object.material;_renderData.elements.push(_line)}function pushTriangle(a,b,c){var v1=_vertexPool[a];var v2=_vertexPool[b];var v3=_vertexPool[c];if(checkTriangleVisibility(v1,v2,v3)===false){return}if(material.side===THREE.DoubleSide||checkBackfaceCulling(v1,v2,v3)===true){_face=getNextFaceInPool();_face.id=object.id;_face.v1.copy(v1);_face.v2.copy(v2);_face.v3.copy(v3);_face.z=(v1.positionScreen.z+v2.positionScreen.z+v3.positionScreen.z)/3;_face.renderOrder=object.renderOrder;_face.normalModel.fromArray(normals,a*3);_face.normalModel.applyMatrix3(normalMatrix).normalize();for(var i=0;i<3;i++){var normal=_face.vertexNormalsModel[i];normal.fromArray(normals,arguments[i]*3);normal.applyMatrix3(normalMatrix).normalize();var uv=_face.uvs[i];uv.fromArray(uvs,arguments[i]*2)}_face.vertexNormalsLength=3;_face.material=object.material;_renderData.elements.push(_face)}}return{setObject:setObject,projectVertex:projectVertex,checkTriangleVisibility:checkTriangleVisibility,checkBackfaceCulling:checkBackfaceCulling,pushVertex:pushVertex,pushNormal:pushNormal,pushUv:pushUv,pushLine:pushLine,pushTriangle:pushTriangle}};var renderList=new RenderList();this.projectScene=function(scene,camera,sortObjects,sortElements){_faceCount=0;_lineCount=0;_spriteCount=0;_renderData.elements.length=0;if(scene.autoUpdate===true){scene.updateMatrixWorld()}if(camera.parent===null){camera.updateMatrixWorld()}_viewMatrix.copy(camera.matrixWorldInverse.getInverse(camera.matrixWorld));_viewProjectionMatrix.multiplyMatrices(camera.projectionMatrix,_viewMatrix);_frustum.setFromMatrix(_viewProjectionMatrix);_objectCount=0;_renderData.objects.length=0;_renderData.lights.length=0;scene.traverseVisible(function(object){if(object instanceof THREE.Light){_renderData.lights.push(object)}else{if(object instanceof THREE.Mesh||object instanceof THREE.Line||object instanceof THREE.Sprite){var material=object.material;if(material.visible===false){return}if(object.frustumCulled===false||_frustum.intersectsObject(object)===true){_object=getNextObjectInPool();_object.id=object.id;_object.object=object;_vector3.setFromMatrixPosition(object.matrixWorld);_vector3.applyProjection(_viewProjectionMatrix);_object.z=_vector3.z;_object.renderOrder=object.renderOrder;_renderData.objects.push(_object)}}}});if(sortObjects===true){_renderData.objects.sort(painterSort)}for(var o=0,ol=_renderData.objects.length;o0){for(var o=0;o0){continue}v2=_vertexPool[_vertexCount-2];_clippedVertex1PositionScreen.copy(v1.positionScreen);_clippedVertex2PositionScreen.copy(v2.positionScreen);if(clipLine(_clippedVertex1PositionScreen,_clippedVertex2PositionScreen)===true){_clippedVertex1PositionScreen.multiplyScalar(1/_clippedVertex1PositionScreen.w);_clippedVertex2PositionScreen.multiplyScalar(1/_clippedVertex2PositionScreen.w);_line=getNextLineInPool();_line.id=object.id;_line.v1.positionScreen.copy(_clippedVertex1PositionScreen);_line.v2.positionScreen.copy(_clippedVertex2PositionScreen);_line.z=Math.max(_clippedVertex1PositionScreen.z,_clippedVertex2PositionScreen.z);_line.renderOrder=object.renderOrder;_line.material=object.material;if(object.material.vertexColors===THREE.VertexColors){_line.vertexColors[0].copy(object.geometry.colors[v]);_line.vertexColors[1].copy(object.geometry.colors[v-1])}_renderData.elements.push(_line)}}}}}else{if(object instanceof THREE.Sprite){_vector4.set(_modelMatrix.elements[12],_modelMatrix.elements[13],_modelMatrix.elements[14],1);_vector4.applyMatrix4(_viewProjectionMatrix);var invW=1/_vector4.w;_vector4.z*=invW;if(_vector4.z>=-1&&_vector4.z<=1){_sprite=getNextSpriteInPool();_sprite.id=object.id;_sprite.x=_vector4.x*invW;_sprite.y=_vector4.y*invW;_sprite.z=_vector4.z;_sprite.renderOrder=object.renderOrder;_sprite.object=object;_sprite.rotation=object.rotation;_sprite.scale.x=object.scale.x*Math.abs(_sprite.x-(_vector4.x+camera.projectionMatrix.elements[0])/(_vector4.w+camera.projectionMatrix.elements[12]));_sprite.scale.y=object.scale.y*Math.abs(_sprite.y-(_vector4.y+camera.projectionMatrix.elements[5])/(_vector4.w+camera.projectionMatrix.elements[13]));_sprite.material=object.material;_renderData.elements.push(_sprite)}}}}}if(sortElements===true){_renderData.elements.sort(painterSort)}return _renderData};function getNextObjectInPool(){if(_objectCount===_objectPoolLength){var object=new THREE.RenderableObject();_objectPool.push(object);_objectPoolLength++;_objectCount++;return object}return _objectPool[_objectCount++]}function getNextVertexInPool(){if(_vertexCount===_vertexPoolLength){var vertex=new THREE.RenderableVertex();_vertexPool.push(vertex);_vertexPoolLength++;_vertexCount++;return vertex}return _vertexPool[_vertexCount++]}function getNextFaceInPool(){if(_faceCount===_facePoolLength){var face=new THREE.RenderableFace();_facePool.push(face);_facePoolLength++;_faceCount++;return face}return _facePool[_faceCount++]}function getNextLineInPool(){if(_lineCount===_linePoolLength){var line=new THREE.RenderableLine();_linePool.push(line);_linePoolLength++;_lineCount++;return line}return _linePool[_lineCount++]}function getNextSpriteInPool(){if(_spriteCount===_spritePoolLength){var sprite=new THREE.RenderableSprite();_spritePool.push(sprite);_spritePoolLength++;_spriteCount++;return sprite}return _spritePool[_spriteCount++]}function painterSort(a,b){if(a.renderOrder!==b.renderOrder){return a.renderOrder-b.renderOrder}else{if(a.z!==b.z){return b.z-a.z}else{if(a.id!==b.id){return a.id-b.id}else{return 0}}}}function clipLine(s1,s2){var alpha1=0,alpha2=1,bc1near=s1.z+s1.w,bc2near=s2.z+s2.w,bc1far=-s1.z+s1.w,bc2far=-s2.z+s2.w;if(bc1near>=0&&bc2near>=0&&bc1far>=0&&bc2far>=0){return true}else{if((bc1near<0&&bc2near<0)||(bc1far<0&&bc2far<0)){return false}else{if(bc1near<0){alpha1=Math.max(alpha1,bc1near/(bc1near-bc2near))}else{if(bc2near<0){alpha2=Math.min(alpha2,bc1near/(bc1near-bc2near))}}if(bc1far<0){alpha1=Math.max(alpha1,bc1far/(bc1far-bc2far))}else{if(bc2far<0){alpha2=Math.min(alpha2,bc1far/(bc1far-bc2far))}}if(alpha2workers){pool.splice(pool.indexOf(this),1);return this.terminate()}renderNext(this)}};worker.color=new THREE.Color().setHSL(Math.random(),0.8,0.8).getHexString();pool.push(worker);if(renderering){updateSettings(worker);worker.postMessage({scene:sceneJSON,camera:cameraJSON,annex:materials,sceneId:sceneId});renderNext(worker)}}if(!renderering){while(pool.length>workers){pool.pop().terminate()}}};this.setWorkers(workers);this.setClearColor=function(color,alpha){clearColor.set(color)};this.setPixelRatio=function(){};this.setSize=function(width,height){canvas.width=width;canvas.height=height;canvasWidth=canvas.width;canvasHeight=canvas.height;canvasWidthHalf=Math.floor(canvasWidth/2);canvasHeightHalf=Math.floor(canvasHeight/2);context.fillStyle="white";pool.forEach(updateSettings)};this.setSize(canvas.width,canvas.height);this.clear=function(){};var totalBlocks,xblocks,yblocks;function updateSettings(worker){worker.postMessage({init:[canvasWidth,canvasHeight],worker:worker.id,blockSize:blockSize})}function renderNext(worker){if(!toRender.length){renderering=false;return scope.dispatchEvent({type:"complete"})}var current=toRender.pop();var blockX=(current%xblocks)*blockSize;var blockY=(current/xblocks|0)*blockSize;worker.postMessage({render:true,x:blockX,y:blockY,sceneId:sceneId});context.fillStyle="#"+worker.color;context.fillRect(blockX,blockY,blockSize,blockSize)}var materials={};var sceneJSON,cameraJSON,reallyThen;var _annex={mirror:1,reflectivity:1,refractionRatio:1,glass:1};function serializeObject(o){var mat=o.material;if(!mat||mat.uuid in materials){return}var props={};for(var m in _annex){if(mat[m]!==undefined){props[m]=mat[m]}}materials[mat.uuid]=props}this.render=function(scene,camera){renderering=true;if(scene.autoUpdate===true){scene.updateMatrixWorld()}if(camera.parent===null){camera.updateMatrixWorld()}sceneJSON=scene.toJSON();cameraJSON=camera.toJSON();++sceneId;scene.traverse(serializeObject);pool.forEach(function(worker){worker.postMessage({scene:sceneJSON,camera:cameraJSON,annex:materials,sceneId:sceneId})});context.clearRect(0,0,canvasWidth,canvasHeight);reallyThen=Date.now();xblocks=Math.ceil(canvasWidth/blockSize);yblocks=Math.ceil(canvasHeight/blockSize);totalBlocks=xblocks*yblocks;toRender=[];for(var i=0;i1?1:elapsed;value=_easingFunction(elapsed);for(property in _valuesEnd){var start=_valuesStart[property]||0;var end=_valuesEnd[property];if(end instanceof Array){_object[property]=_interpolationFunction(end,value)}else{if(typeof(end)==="string"){end=start+parseFloat(end,10)}if(typeof(end)==="number"){_object[property]=start+(end-start)*value}}}if(_onUpdateCallback!==null){_onUpdateCallback.call(_object,value)}if(elapsed===1){if(_repeat>0){if(isFinite(_repeat)){_repeat--}for(property in _valuesStartRepeat){if(typeof(_valuesEnd[property])==="string"){_valuesStartRepeat[property]=_valuesStartRepeat[property]+parseFloat(_valuesEnd[property],10)}if(_yoyo){var tmp=_valuesStartRepeat[property];_valuesStartRepeat[property]=_valuesEnd[property];_valuesEnd[property]=tmp}_valuesStart[property]=_valuesStartRepeat[property]}if(_yoyo){_reversed=!_reversed}_startTime=time+_delayTime;return true}else{if(_onCompleteCallback!==null){_onCompleteCallback.call(_object)}for(var i=0,numChainedTweens=_chainedTweens.length;i1){return fn(v[m],v[m-1],m-f)}return fn(v[i],v[i+1>m?m:i+1],f-i)},Bezier:function(v,k){var b=0;var n=v.length-1;var pw=Math.pow;var bn=TWEEN.Interpolation.Utils.Bernstein;for(var i=0;i<=n;i++){b+=pw(1-k,n-i)*pw(k,i)*v[i]*bn(n,i)}return b},CatmullRom:function(v,k){var m=v.length-1;var f=m*k;var i=Math.floor(f);var fn=TWEEN.Interpolation.Utils.CatmullRom;if(v[0]===v[m]){if(k<0){i=Math.floor(f=m*(1+k))}return fn(v[(i-1+m)%m],v[i],v[(i+1)%m],v[(i+2)%m],f-i)}else{if(k<0){return v[0]-(fn(v[0],v[0],v[1],v[1],-f)-v[0])}if(k>1){return v[m]-(fn(v[m],v[m],v[m-1],v[m-1],f-m)-v[m])}return fn(v[i?i-1:0],v[i],v[m1;i--){s*=i}a[n]=s;return s}})(),CatmullRom:function(p0,p1,p2,p3,t){var v0=(p2-p0)*0.5;var v1=(p3-p1)*0.5;var t2=t*t;var t3=t*t2;return(2*p1-2*p2+v0+v1)*t3+(-3*p1+3*p2-2*v0-v1)*t2+v0*t+p1}}};(function(root){if(typeof define==="function"&&define.amd){define([],function(){return TWEEN})}else{if(typeof exports==="object"){module.exports=TWEEN}else{root.TWEEN=TWEEN}}})(this);
\ No newline at end of file
diff --git a/js/jquery-2.1.4.min.js b/js/jquery-2.1.4.min.js
new file mode 100644
index 0000000..49990d6
--- /dev/null
+++ b/js/jquery-2.1.4.min.js
@@ -0,0 +1,4 @@
+/*! jQuery v2.1.4 | (c) 2005, 2015 jQuery Foundation, Inc. | jquery.org/license */
+!function(a,b){"object"==typeof module&&"object"==typeof module.exports?module.exports=a.document?b(a,!0):function(a){if(!a.document)throw new Error("jQuery requires a window with a document");return b(a)}:b(a)}("undefined"!=typeof window?window:this,function(a,b){var c=[],d=c.slice,e=c.concat,f=c.push,g=c.indexOf,h={},i=h.toString,j=h.hasOwnProperty,k={},l=a.document,m="2.1.4",n=function(a,b){return new n.fn.init(a,b)},o=/^[\s\uFEFF\xA0]+|[\s\uFEFF\xA0]+$/g,p=/^-ms-/,q=/-([\da-z])/gi,r=function(a,b){return b.toUpperCase()};n.fn=n.prototype={jquery:m,constructor:n,selector:"",length:0,toArray:function(){return d.call(this)},get:function(a){return null!=a?0>a?this[a+this.length]:this[a]:d.call(this)},pushStack:function(a){var b=n.merge(this.constructor(),a);return b.prevObject=this,b.context=this.context,b},each:function(a,b){return n.each(this,a,b)},map:function(a){return this.pushStack(n.map(this,function(b,c){return a.call(b,c,b)}))},slice:function(){return this.pushStack(d.apply(this,arguments))},first:function(){return this.eq(0)},last:function(){return this.eq(-1)},eq:function(a){var b=this.length,c=+a+(0>a?b:0);return this.pushStack(c>=0&&b>c?[this[c]]:[])},end:function(){return this.prevObject||this.constructor(null)},push:f,sort:c.sort,splice:c.splice},n.extend=n.fn.extend=function(){var a,b,c,d,e,f,g=arguments[0]||{},h=1,i=arguments.length,j=!1;for("boolean"==typeof g&&(j=g,g=arguments[h]||{},h++),"object"==typeof g||n.isFunction(g)||(g={}),h===i&&(g=this,h--);i>h;h++)if(null!=(a=arguments[h]))for(b in a)c=g[b],d=a[b],g!==d&&(j&&d&&(n.isPlainObject(d)||(e=n.isArray(d)))?(e?(e=!1,f=c&&n.isArray(c)?c:[]):f=c&&n.isPlainObject(c)?c:{},g[b]=n.extend(j,f,d)):void 0!==d&&(g[b]=d));return g},n.extend({expando:"jQuery"+(m+Math.random()).replace(/\D/g,""),isReady:!0,error:function(a){throw new Error(a)},noop:function(){},isFunction:function(a){return"function"===n.type(a)},isArray:Array.isArray,isWindow:function(a){return null!=a&&a===a.window},isNumeric:function(a){return!n.isArray(a)&&a-parseFloat(a)+1>=0},isPlainObject:function(a){return"object"!==n.type(a)||a.nodeType||n.isWindow(a)?!1:a.constructor&&!j.call(a.constructor.prototype,"isPrototypeOf")?!1:!0},isEmptyObject:function(a){var b;for(b in a)return!1;return!0},type:function(a){return null==a?a+"":"object"==typeof a||"function"==typeof a?h[i.call(a)]||"object":typeof a},globalEval:function(a){var b,c=eval;a=n.trim(a),a&&(1===a.indexOf("use strict")?(b=l.createElement("script"),b.text=a,l.head.appendChild(b).parentNode.removeChild(b)):c(a))},camelCase:function(a){return a.replace(p,"ms-").replace(q,r)},nodeName:function(a,b){return a.nodeName&&a.nodeName.toLowerCase()===b.toLowerCase()},each:function(a,b,c){var d,e=0,f=a.length,g=s(a);if(c){if(g){for(;f>e;e++)if(d=b.apply(a[e],c),d===!1)break}else for(e in a)if(d=b.apply(a[e],c),d===!1)break}else if(g){for(;f>e;e++)if(d=b.call(a[e],e,a[e]),d===!1)break}else for(e in a)if(d=b.call(a[e],e,a[e]),d===!1)break;return a},trim:function(a){return null==a?"":(a+"").replace(o,"")},makeArray:function(a,b){var c=b||[];return null!=a&&(s(Object(a))?n.merge(c,"string"==typeof a?[a]:a):f.call(c,a)),c},inArray:function(a,b,c){return null==b?-1:g.call(b,a,c)},merge:function(a,b){for(var c=+b.length,d=0,e=a.length;c>d;d++)a[e++]=b[d];return a.length=e,a},grep:function(a,b,c){for(var d,e=[],f=0,g=a.length,h=!c;g>f;f++)d=!b(a[f],f),d!==h&&e.push(a[f]);return e},map:function(a,b,c){var d,f=0,g=a.length,h=s(a),i=[];if(h)for(;g>f;f++)d=b(a[f],f,c),null!=d&&i.push(d);else for(f in a)d=b(a[f],f,c),null!=d&&i.push(d);return e.apply([],i)},guid:1,proxy:function(a,b){var c,e,f;return"string"==typeof b&&(c=a[b],b=a,a=c),n.isFunction(a)?(e=d.call(arguments,2),f=function(){return a.apply(b||this,e.concat(d.call(arguments)))},f.guid=a.guid=a.guid||n.guid++,f):void 0},now:Date.now,support:k}),n.each("Boolean Number String Function Array Date RegExp Object Error".split(" "),function(a,b){h["[object "+b+"]"]=b.toLowerCase()});function s(a){var b="length"in a&&a.length,c=n.type(a);return"function"===c||n.isWindow(a)?!1:1===a.nodeType&&b?!0:"array"===c||0===b||"number"==typeof b&&b>0&&b-1 in a}var t=function(a){var b,c,d,e,f,g,h,i,j,k,l,m,n,o,p,q,r,s,t,u="sizzle"+1*new Date,v=a.document,w=0,x=0,y=ha(),z=ha(),A=ha(),B=function(a,b){return a===b&&(l=!0),0},C=1<<31,D={}.hasOwnProperty,E=[],F=E.pop,G=E.push,H=E.push,I=E.slice,J=function(a,b){for(var c=0,d=a.length;d>c;c++)if(a[c]===b)return c;return-1},K="checked|selected|async|autofocus|autoplay|controls|defer|disabled|hidden|ismap|loop|multiple|open|readonly|required|scoped",L="[\\x20\\t\\r\\n\\f]",M="(?:\\\\.|[\\w-]|[^\\x00-\\xa0])+",N=M.replace("w","w#"),O="\\["+L+"*("+M+")(?:"+L+"*([*^$|!~]?=)"+L+"*(?:'((?:\\\\.|[^\\\\'])*)'|\"((?:\\\\.|[^\\\\\"])*)\"|("+N+"))|)"+L+"*\\]",P=":("+M+")(?:\\((('((?:\\\\.|[^\\\\'])*)'|\"((?:\\\\.|[^\\\\\"])*)\")|((?:\\\\.|[^\\\\()[\\]]|"+O+")*)|.*)\\)|)",Q=new RegExp(L+"+","g"),R=new RegExp("^"+L+"+|((?:^|[^\\\\])(?:\\\\.)*)"+L+"+$","g"),S=new RegExp("^"+L+"*,"+L+"*"),T=new RegExp("^"+L+"*([>+~]|"+L+")"+L+"*"),U=new RegExp("="+L+"*([^\\]'\"]*?)"+L+"*\\]","g"),V=new RegExp(P),W=new RegExp("^"+N+"$"),X={ID:new RegExp("^#("+M+")"),CLASS:new RegExp("^\\.("+M+")"),TAG:new RegExp("^("+M.replace("w","w*")+")"),ATTR:new RegExp("^"+O),PSEUDO:new RegExp("^"+P),CHILD:new RegExp("^:(only|first|last|nth|nth-last)-(child|of-type)(?:\\("+L+"*(even|odd|(([+-]|)(\\d*)n|)"+L+"*(?:([+-]|)"+L+"*(\\d+)|))"+L+"*\\)|)","i"),bool:new RegExp("^(?:"+K+")$","i"),needsContext:new RegExp("^"+L+"*[>+~]|:(even|odd|eq|gt|lt|nth|first|last)(?:\\("+L+"*((?:-\\d)?\\d*)"+L+"*\\)|)(?=[^-]|$)","i")},Y=/^(?:input|select|textarea|button)$/i,Z=/^h\d$/i,$=/^[^{]+\{\s*\[native \w/,_=/^(?:#([\w-]+)|(\w+)|\.([\w-]+))$/,aa=/[+~]/,ba=/'|\\/g,ca=new RegExp("\\\\([\\da-f]{1,6}"+L+"?|("+L+")|.)","ig"),da=function(a,b,c){var d="0x"+b-65536;return d!==d||c?b:0>d?String.fromCharCode(d+65536):String.fromCharCode(d>>10|55296,1023&d|56320)},ea=function(){m()};try{H.apply(E=I.call(v.childNodes),v.childNodes),E[v.childNodes.length].nodeType}catch(fa){H={apply:E.length?function(a,b){G.apply(a,I.call(b))}:function(a,b){var c=a.length,d=0;while(a[c++]=b[d++]);a.length=c-1}}}function ga(a,b,d,e){var f,h,j,k,l,o,r,s,w,x;if((b?b.ownerDocument||b:v)!==n&&m(b),b=b||n,d=d||[],k=b.nodeType,"string"!=typeof a||!a||1!==k&&9!==k&&11!==k)return d;if(!e&&p){if(11!==k&&(f=_.exec(a)))if(j=f[1]){if(9===k){if(h=b.getElementById(j),!h||!h.parentNode)return d;if(h.id===j)return d.push(h),d}else if(b.ownerDocument&&(h=b.ownerDocument.getElementById(j))&&t(b,h)&&h.id===j)return d.push(h),d}else{if(f[2])return H.apply(d,b.getElementsByTagName(a)),d;if((j=f[3])&&c.getElementsByClassName)return H.apply(d,b.getElementsByClassName(j)),d}if(c.qsa&&(!q||!q.test(a))){if(s=r=u,w=b,x=1!==k&&a,1===k&&"object"!==b.nodeName.toLowerCase()){o=g(a),(r=b.getAttribute("id"))?s=r.replace(ba,"\\$&"):b.setAttribute("id",s),s="[id='"+s+"'] ",l=o.length;while(l--)o[l]=s+ra(o[l]);w=aa.test(a)&&pa(b.parentNode)||b,x=o.join(",")}if(x)try{return H.apply(d,w.querySelectorAll(x)),d}catch(y){}finally{r||b.removeAttribute("id")}}}return i(a.replace(R,"$1"),b,d,e)}function ha(){var a=[];function b(c,e){return a.push(c+" ")>d.cacheLength&&delete b[a.shift()],b[c+" "]=e}return b}function ia(a){return a[u]=!0,a}function ja(a){var b=n.createElement("div");try{return!!a(b)}catch(c){return!1}finally{b.parentNode&&b.parentNode.removeChild(b),b=null}}function ka(a,b){var c=a.split("|"),e=a.length;while(e--)d.attrHandle[c[e]]=b}function la(a,b){var c=b&&a,d=c&&1===a.nodeType&&1===b.nodeType&&(~b.sourceIndex||C)-(~a.sourceIndex||C);if(d)return d;if(c)while(c=c.nextSibling)if(c===b)return-1;return a?1:-1}function ma(a){return function(b){var c=b.nodeName.toLowerCase();return"input"===c&&b.type===a}}function na(a){return function(b){var c=b.nodeName.toLowerCase();return("input"===c||"button"===c)&&b.type===a}}function oa(a){return ia(function(b){return b=+b,ia(function(c,d){var e,f=a([],c.length,b),g=f.length;while(g--)c[e=f[g]]&&(c[e]=!(d[e]=c[e]))})})}function pa(a){return a&&"undefined"!=typeof a.getElementsByTagName&&a}c=ga.support={},f=ga.isXML=function(a){var b=a&&(a.ownerDocument||a).documentElement;return b?"HTML"!==b.nodeName:!1},m=ga.setDocument=function(a){var b,e,g=a?a.ownerDocument||a:v;return g!==n&&9===g.nodeType&&g.documentElement?(n=g,o=g.documentElement,e=g.defaultView,e&&e!==e.top&&(e.addEventListener?e.addEventListener("unload",ea,!1):e.attachEvent&&e.attachEvent("onunload",ea)),p=!f(g),c.attributes=ja(function(a){return a.className="i",!a.getAttribute("className")}),c.getElementsByTagName=ja(function(a){return a.appendChild(g.createComment("")),!a.getElementsByTagName("*").length}),c.getElementsByClassName=$.test(g.getElementsByClassName),c.getById=ja(function(a){return o.appendChild(a).id=u,!g.getElementsByName||!g.getElementsByName(u).length}),c.getById?(d.find.ID=function(a,b){if("undefined"!=typeof b.getElementById&&p){var c=b.getElementById(a);return c&&c.parentNode?[c]:[]}},d.filter.ID=function(a){var b=a.replace(ca,da);return function(a){return a.getAttribute("id")===b}}):(delete d.find.ID,d.filter.ID=function(a){var b=a.replace(ca,da);return function(a){var c="undefined"!=typeof a.getAttributeNode&&a.getAttributeNode("id");return c&&c.value===b}}),d.find.TAG=c.getElementsByTagName?function(a,b){return"undefined"!=typeof b.getElementsByTagName?b.getElementsByTagName(a):c.qsa?b.querySelectorAll(a):void 0}:function(a,b){var c,d=[],e=0,f=b.getElementsByTagName(a);if("*"===a){while(c=f[e++])1===c.nodeType&&d.push(c);return d}return f},d.find.CLASS=c.getElementsByClassName&&function(a,b){return p?b.getElementsByClassName(a):void 0},r=[],q=[],(c.qsa=$.test(g.querySelectorAll))&&(ja(function(a){o.appendChild(a).innerHTML="",a.querySelectorAll("[msallowcapture^='']").length&&q.push("[*^$]="+L+"*(?:''|\"\")"),a.querySelectorAll("[selected]").length||q.push("\\["+L+"*(?:value|"+K+")"),a.querySelectorAll("[id~="+u+"-]").length||q.push("~="),a.querySelectorAll(":checked").length||q.push(":checked"),a.querySelectorAll("a#"+u+"+*").length||q.push(".#.+[+~]")}),ja(function(a){var b=g.createElement("input");b.setAttribute("type","hidden"),a.appendChild(b).setAttribute("name","D"),a.querySelectorAll("[name=d]").length&&q.push("name"+L+"*[*^$|!~]?="),a.querySelectorAll(":enabled").length||q.push(":enabled",":disabled"),a.querySelectorAll("*,:x"),q.push(",.*:")})),(c.matchesSelector=$.test(s=o.matches||o.webkitMatchesSelector||o.mozMatchesSelector||o.oMatchesSelector||o.msMatchesSelector))&&ja(function(a){c.disconnectedMatch=s.call(a,"div"),s.call(a,"[s!='']:x"),r.push("!=",P)}),q=q.length&&new RegExp(q.join("|")),r=r.length&&new RegExp(r.join("|")),b=$.test(o.compareDocumentPosition),t=b||$.test(o.contains)?function(a,b){var c=9===a.nodeType?a.documentElement:a,d=b&&b.parentNode;return a===d||!(!d||1!==d.nodeType||!(c.contains?c.contains(d):a.compareDocumentPosition&&16&a.compareDocumentPosition(d)))}:function(a,b){if(b)while(b=b.parentNode)if(b===a)return!0;return!1},B=b?function(a,b){if(a===b)return l=!0,0;var d=!a.compareDocumentPosition-!b.compareDocumentPosition;return d?d:(d=(a.ownerDocument||a)===(b.ownerDocument||b)?a.compareDocumentPosition(b):1,1&d||!c.sortDetached&&b.compareDocumentPosition(a)===d?a===g||a.ownerDocument===v&&t(v,a)?-1:b===g||b.ownerDocument===v&&t(v,b)?1:k?J(k,a)-J(k,b):0:4&d?-1:1)}:function(a,b){if(a===b)return l=!0,0;var c,d=0,e=a.parentNode,f=b.parentNode,h=[a],i=[b];if(!e||!f)return a===g?-1:b===g?1:e?-1:f?1:k?J(k,a)-J(k,b):0;if(e===f)return la(a,b);c=a;while(c=c.parentNode)h.unshift(c);c=b;while(c=c.parentNode)i.unshift(c);while(h[d]===i[d])d++;return d?la(h[d],i[d]):h[d]===v?-1:i[d]===v?1:0},g):n},ga.matches=function(a,b){return ga(a,null,null,b)},ga.matchesSelector=function(a,b){if((a.ownerDocument||a)!==n&&m(a),b=b.replace(U,"='$1']"),!(!c.matchesSelector||!p||r&&r.test(b)||q&&q.test(b)))try{var d=s.call(a,b);if(d||c.disconnectedMatch||a.document&&11!==a.document.nodeType)return d}catch(e){}return ga(b,n,null,[a]).length>0},ga.contains=function(a,b){return(a.ownerDocument||a)!==n&&m(a),t(a,b)},ga.attr=function(a,b){(a.ownerDocument||a)!==n&&m(a);var e=d.attrHandle[b.toLowerCase()],f=e&&D.call(d.attrHandle,b.toLowerCase())?e(a,b,!p):void 0;return void 0!==f?f:c.attributes||!p?a.getAttribute(b):(f=a.getAttributeNode(b))&&f.specified?f.value:null},ga.error=function(a){throw new Error("Syntax error, unrecognized expression: "+a)},ga.uniqueSort=function(a){var b,d=[],e=0,f=0;if(l=!c.detectDuplicates,k=!c.sortStable&&a.slice(0),a.sort(B),l){while(b=a[f++])b===a[f]&&(e=d.push(f));while(e--)a.splice(d[e],1)}return k=null,a},e=ga.getText=function(a){var b,c="",d=0,f=a.nodeType;if(f){if(1===f||9===f||11===f){if("string"==typeof a.textContent)return a.textContent;for(a=a.firstChild;a;a=a.nextSibling)c+=e(a)}else if(3===f||4===f)return a.nodeValue}else while(b=a[d++])c+=e(b);return c},d=ga.selectors={cacheLength:50,createPseudo:ia,match:X,attrHandle:{},find:{},relative:{">":{dir:"parentNode",first:!0}," ":{dir:"parentNode"},"+":{dir:"previousSibling",first:!0},"~":{dir:"previousSibling"}},preFilter:{ATTR:function(a){return a[1]=a[1].replace(ca,da),a[3]=(a[3]||a[4]||a[5]||"").replace(ca,da),"~="===a[2]&&(a[3]=" "+a[3]+" "),a.slice(0,4)},CHILD:function(a){return a[1]=a[1].toLowerCase(),"nth"===a[1].slice(0,3)?(a[3]||ga.error(a[0]),a[4]=+(a[4]?a[5]+(a[6]||1):2*("even"===a[3]||"odd"===a[3])),a[5]=+(a[7]+a[8]||"odd"===a[3])):a[3]&&ga.error(a[0]),a},PSEUDO:function(a){var b,c=!a[6]&&a[2];return X.CHILD.test(a[0])?null:(a[3]?a[2]=a[4]||a[5]||"":c&&V.test(c)&&(b=g(c,!0))&&(b=c.indexOf(")",c.length-b)-c.length)&&(a[0]=a[0].slice(0,b),a[2]=c.slice(0,b)),a.slice(0,3))}},filter:{TAG:function(a){var b=a.replace(ca,da).toLowerCase();return"*"===a?function(){return!0}:function(a){return a.nodeName&&a.nodeName.toLowerCase()===b}},CLASS:function(a){var b=y[a+" "];return b||(b=new RegExp("(^|"+L+")"+a+"("+L+"|$)"))&&y(a,function(a){return b.test("string"==typeof a.className&&a.className||"undefined"!=typeof a.getAttribute&&a.getAttribute("class")||"")})},ATTR:function(a,b,c){return function(d){var e=ga.attr(d,a);return null==e?"!="===b:b?(e+="","="===b?e===c:"!="===b?e!==c:"^="===b?c&&0===e.indexOf(c):"*="===b?c&&e.indexOf(c)>-1:"$="===b?c&&e.slice(-c.length)===c:"~="===b?(" "+e.replace(Q," ")+" ").indexOf(c)>-1:"|="===b?e===c||e.slice(0,c.length+1)===c+"-":!1):!0}},CHILD:function(a,b,c,d,e){var f="nth"!==a.slice(0,3),g="last"!==a.slice(-4),h="of-type"===b;return 1===d&&0===e?function(a){return!!a.parentNode}:function(b,c,i){var j,k,l,m,n,o,p=f!==g?"nextSibling":"previousSibling",q=b.parentNode,r=h&&b.nodeName.toLowerCase(),s=!i&&!h;if(q){if(f){while(p){l=b;while(l=l[p])if(h?l.nodeName.toLowerCase()===r:1===l.nodeType)return!1;o=p="only"===a&&!o&&"nextSibling"}return!0}if(o=[g?q.firstChild:q.lastChild],g&&s){k=q[u]||(q[u]={}),j=k[a]||[],n=j[0]===w&&j[1],m=j[0]===w&&j[2],l=n&&q.childNodes[n];while(l=++n&&l&&l[p]||(m=n=0)||o.pop())if(1===l.nodeType&&++m&&l===b){k[a]=[w,n,m];break}}else if(s&&(j=(b[u]||(b[u]={}))[a])&&j[0]===w)m=j[1];else while(l=++n&&l&&l[p]||(m=n=0)||o.pop())if((h?l.nodeName.toLowerCase()===r:1===l.nodeType)&&++m&&(s&&((l[u]||(l[u]={}))[a]=[w,m]),l===b))break;return m-=e,m===d||m%d===0&&m/d>=0}}},PSEUDO:function(a,b){var c,e=d.pseudos[a]||d.setFilters[a.toLowerCase()]||ga.error("unsupported pseudo: "+a);return e[u]?e(b):e.length>1?(c=[a,a,"",b],d.setFilters.hasOwnProperty(a.toLowerCase())?ia(function(a,c){var d,f=e(a,b),g=f.length;while(g--)d=J(a,f[g]),a[d]=!(c[d]=f[g])}):function(a){return e(a,0,c)}):e}},pseudos:{not:ia(function(a){var b=[],c=[],d=h(a.replace(R,"$1"));return d[u]?ia(function(a,b,c,e){var f,g=d(a,null,e,[]),h=a.length;while(h--)(f=g[h])&&(a[h]=!(b[h]=f))}):function(a,e,f){return b[0]=a,d(b,null,f,c),b[0]=null,!c.pop()}}),has:ia(function(a){return function(b){return ga(a,b).length>0}}),contains:ia(function(a){return a=a.replace(ca,da),function(b){return(b.textContent||b.innerText||e(b)).indexOf(a)>-1}}),lang:ia(function(a){return W.test(a||"")||ga.error("unsupported lang: "+a),a=a.replace(ca,da).toLowerCase(),function(b){var c;do if(c=p?b.lang:b.getAttribute("xml:lang")||b.getAttribute("lang"))return c=c.toLowerCase(),c===a||0===c.indexOf(a+"-");while((b=b.parentNode)&&1===b.nodeType);return!1}}),target:function(b){var c=a.location&&a.location.hash;return c&&c.slice(1)===b.id},root:function(a){return a===o},focus:function(a){return a===n.activeElement&&(!n.hasFocus||n.hasFocus())&&!!(a.type||a.href||~a.tabIndex)},enabled:function(a){return a.disabled===!1},disabled:function(a){return a.disabled===!0},checked:function(a){var b=a.nodeName.toLowerCase();return"input"===b&&!!a.checked||"option"===b&&!!a.selected},selected:function(a){return a.parentNode&&a.parentNode.selectedIndex,a.selected===!0},empty:function(a){for(a=a.firstChild;a;a=a.nextSibling)if(a.nodeType<6)return!1;return!0},parent:function(a){return!d.pseudos.empty(a)},header:function(a){return Z.test(a.nodeName)},input:function(a){return Y.test(a.nodeName)},button:function(a){var b=a.nodeName.toLowerCase();return"input"===b&&"button"===a.type||"button"===b},text:function(a){var b;return"input"===a.nodeName.toLowerCase()&&"text"===a.type&&(null==(b=a.getAttribute("type"))||"text"===b.toLowerCase())},first:oa(function(){return[0]}),last:oa(function(a,b){return[b-1]}),eq:oa(function(a,b,c){return[0>c?c+b:c]}),even:oa(function(a,b){for(var c=0;b>c;c+=2)a.push(c);return a}),odd:oa(function(a,b){for(var c=1;b>c;c+=2)a.push(c);return a}),lt:oa(function(a,b,c){for(var d=0>c?c+b:c;--d>=0;)a.push(d);return a}),gt:oa(function(a,b,c){for(var d=0>c?c+b:c;++db;b++)d+=a[b].value;return d}function sa(a,b,c){var d=b.dir,e=c&&"parentNode"===d,f=x++;return b.first?function(b,c,f){while(b=b[d])if(1===b.nodeType||e)return a(b,c,f)}:function(b,c,g){var h,i,j=[w,f];if(g){while(b=b[d])if((1===b.nodeType||e)&&a(b,c,g))return!0}else while(b=b[d])if(1===b.nodeType||e){if(i=b[u]||(b[u]={}),(h=i[d])&&h[0]===w&&h[1]===f)return j[2]=h[2];if(i[d]=j,j[2]=a(b,c,g))return!0}}}function ta(a){return a.length>1?function(b,c,d){var e=a.length;while(e--)if(!a[e](b,c,d))return!1;return!0}:a[0]}function ua(a,b,c){for(var d=0,e=b.length;e>d;d++)ga(a,b[d],c);return c}function va(a,b,c,d,e){for(var f,g=[],h=0,i=a.length,j=null!=b;i>h;h++)(f=a[h])&&(!c||c(f,d,e))&&(g.push(f),j&&b.push(h));return g}function wa(a,b,c,d,e,f){return d&&!d[u]&&(d=wa(d)),e&&!e[u]&&(e=wa(e,f)),ia(function(f,g,h,i){var j,k,l,m=[],n=[],o=g.length,p=f||ua(b||"*",h.nodeType?[h]:h,[]),q=!a||!f&&b?p:va(p,m,a,h,i),r=c?e||(f?a:o||d)?[]:g:q;if(c&&c(q,r,h,i),d){j=va(r,n),d(j,[],h,i),k=j.length;while(k--)(l=j[k])&&(r[n[k]]=!(q[n[k]]=l))}if(f){if(e||a){if(e){j=[],k=r.length;while(k--)(l=r[k])&&j.push(q[k]=l);e(null,r=[],j,i)}k=r.length;while(k--)(l=r[k])&&(j=e?J(f,l):m[k])>-1&&(f[j]=!(g[j]=l))}}else r=va(r===g?r.splice(o,r.length):r),e?e(null,g,r,i):H.apply(g,r)})}function xa(a){for(var b,c,e,f=a.length,g=d.relative[a[0].type],h=g||d.relative[" "],i=g?1:0,k=sa(function(a){return a===b},h,!0),l=sa(function(a){return J(b,a)>-1},h,!0),m=[function(a,c,d){var e=!g&&(d||c!==j)||((b=c).nodeType?k(a,c,d):l(a,c,d));return b=null,e}];f>i;i++)if(c=d.relative[a[i].type])m=[sa(ta(m),c)];else{if(c=d.filter[a[i].type].apply(null,a[i].matches),c[u]){for(e=++i;f>e;e++)if(d.relative[a[e].type])break;return wa(i>1&&ta(m),i>1&&ra(a.slice(0,i-1).concat({value:" "===a[i-2].type?"*":""})).replace(R,"$1"),c,e>i&&xa(a.slice(i,e)),f>e&&xa(a=a.slice(e)),f>e&&ra(a))}m.push(c)}return ta(m)}function ya(a,b){var c=b.length>0,e=a.length>0,f=function(f,g,h,i,k){var l,m,o,p=0,q="0",r=f&&[],s=[],t=j,u=f||e&&d.find.TAG("*",k),v=w+=null==t?1:Math.random()||.1,x=u.length;for(k&&(j=g!==n&&g);q!==x&&null!=(l=u[q]);q++){if(e&&l){m=0;while(o=a[m++])if(o(l,g,h)){i.push(l);break}k&&(w=v)}c&&((l=!o&&l)&&p--,f&&r.push(l))}if(p+=q,c&&q!==p){m=0;while(o=b[m++])o(r,s,g,h);if(f){if(p>0)while(q--)r[q]||s[q]||(s[q]=F.call(i));s=va(s)}H.apply(i,s),k&&!f&&s.length>0&&p+b.length>1&&ga.uniqueSort(i)}return k&&(w=v,j=t),r};return c?ia(f):f}return h=ga.compile=function(a,b){var c,d=[],e=[],f=A[a+" "];if(!f){b||(b=g(a)),c=b.length;while(c--)f=xa(b[c]),f[u]?d.push(f):e.push(f);f=A(a,ya(e,d)),f.selector=a}return f},i=ga.select=function(a,b,e,f){var i,j,k,l,m,n="function"==typeof a&&a,o=!f&&g(a=n.selector||a);if(e=e||[],1===o.length){if(j=o[0]=o[0].slice(0),j.length>2&&"ID"===(k=j[0]).type&&c.getById&&9===b.nodeType&&p&&d.relative[j[1].type]){if(b=(d.find.ID(k.matches[0].replace(ca,da),b)||[])[0],!b)return e;n&&(b=b.parentNode),a=a.slice(j.shift().value.length)}i=X.needsContext.test(a)?0:j.length;while(i--){if(k=j[i],d.relative[l=k.type])break;if((m=d.find[l])&&(f=m(k.matches[0].replace(ca,da),aa.test(j[0].type)&&pa(b.parentNode)||b))){if(j.splice(i,1),a=f.length&&ra(j),!a)return H.apply(e,f),e;break}}}return(n||h(a,o))(f,b,!p,e,aa.test(a)&&pa(b.parentNode)||b),e},c.sortStable=u.split("").sort(B).join("")===u,c.detectDuplicates=!!l,m(),c.sortDetached=ja(function(a){return 1&a.compareDocumentPosition(n.createElement("div"))}),ja(function(a){return a.innerHTML="","#"===a.firstChild.getAttribute("href")})||ka("type|href|height|width",function(a,b,c){return c?void 0:a.getAttribute(b,"type"===b.toLowerCase()?1:2)}),c.attributes&&ja(function(a){return a.innerHTML="",a.firstChild.setAttribute("value",""),""===a.firstChild.getAttribute("value")})||ka("value",function(a,b,c){return c||"input"!==a.nodeName.toLowerCase()?void 0:a.defaultValue}),ja(function(a){return null==a.getAttribute("disabled")})||ka(K,function(a,b,c){var d;return c?void 0:a[b]===!0?b.toLowerCase():(d=a.getAttributeNode(b))&&d.specified?d.value:null}),ga}(a);n.find=t,n.expr=t.selectors,n.expr[":"]=n.expr.pseudos,n.unique=t.uniqueSort,n.text=t.getText,n.isXMLDoc=t.isXML,n.contains=t.contains;var u=n.expr.match.needsContext,v=/^<(\w+)\s*\/?>(?:<\/\1>|)$/,w=/^.[^:#\[\.,]*$/;function x(a,b,c){if(n.isFunction(b))return n.grep(a,function(a,d){return!!b.call(a,d,a)!==c});if(b.nodeType)return n.grep(a,function(a){return a===b!==c});if("string"==typeof b){if(w.test(b))return n.filter(b,a,c);b=n.filter(b,a)}return n.grep(a,function(a){return g.call(b,a)>=0!==c})}n.filter=function(a,b,c){var d=b[0];return c&&(a=":not("+a+")"),1===b.length&&1===d.nodeType?n.find.matchesSelector(d,a)?[d]:[]:n.find.matches(a,n.grep(b,function(a){return 1===a.nodeType}))},n.fn.extend({find:function(a){var b,c=this.length,d=[],e=this;if("string"!=typeof a)return this.pushStack(n(a).filter(function(){for(b=0;c>b;b++)if(n.contains(e[b],this))return!0}));for(b=0;c>b;b++)n.find(a,e[b],d);return d=this.pushStack(c>1?n.unique(d):d),d.selector=this.selector?this.selector+" "+a:a,d},filter:function(a){return this.pushStack(x(this,a||[],!1))},not:function(a){return this.pushStack(x(this,a||[],!0))},is:function(a){return!!x(this,"string"==typeof a&&u.test(a)?n(a):a||[],!1).length}});var y,z=/^(?:\s*(<[\w\W]+>)[^>]*|#([\w-]*))$/,A=n.fn.init=function(a,b){var c,d;if(!a)return this;if("string"==typeof a){if(c="<"===a[0]&&">"===a[a.length-1]&&a.length>=3?[null,a,null]:z.exec(a),!c||!c[1]&&b)return!b||b.jquery?(b||y).find(a):this.constructor(b).find(a);if(c[1]){if(b=b instanceof n?b[0]:b,n.merge(this,n.parseHTML(c[1],b&&b.nodeType?b.ownerDocument||b:l,!0)),v.test(c[1])&&n.isPlainObject(b))for(c in b)n.isFunction(this[c])?this[c](b[c]):this.attr(c,b[c]);return this}return d=l.getElementById(c[2]),d&&d.parentNode&&(this.length=1,this[0]=d),this.context=l,this.selector=a,this}return a.nodeType?(this.context=this[0]=a,this.length=1,this):n.isFunction(a)?"undefined"!=typeof y.ready?y.ready(a):a(n):(void 0!==a.selector&&(this.selector=a.selector,this.context=a.context),n.makeArray(a,this))};A.prototype=n.fn,y=n(l);var B=/^(?:parents|prev(?:Until|All))/,C={children:!0,contents:!0,next:!0,prev:!0};n.extend({dir:function(a,b,c){var d=[],e=void 0!==c;while((a=a[b])&&9!==a.nodeType)if(1===a.nodeType){if(e&&n(a).is(c))break;d.push(a)}return d},sibling:function(a,b){for(var c=[];a;a=a.nextSibling)1===a.nodeType&&a!==b&&c.push(a);return c}}),n.fn.extend({has:function(a){var b=n(a,this),c=b.length;return this.filter(function(){for(var a=0;c>a;a++)if(n.contains(this,b[a]))return!0})},closest:function(a,b){for(var c,d=0,e=this.length,f=[],g=u.test(a)||"string"!=typeof a?n(a,b||this.context):0;e>d;d++)for(c=this[d];c&&c!==b;c=c.parentNode)if(c.nodeType<11&&(g?g.index(c)>-1:1===c.nodeType&&n.find.matchesSelector(c,a))){f.push(c);break}return this.pushStack(f.length>1?n.unique(f):f)},index:function(a){return a?"string"==typeof a?g.call(n(a),this[0]):g.call(this,a.jquery?a[0]:a):this[0]&&this[0].parentNode?this.first().prevAll().length:-1},add:function(a,b){return this.pushStack(n.unique(n.merge(this.get(),n(a,b))))},addBack:function(a){return this.add(null==a?this.prevObject:this.prevObject.filter(a))}});function D(a,b){while((a=a[b])&&1!==a.nodeType);return a}n.each({parent:function(a){var b=a.parentNode;return b&&11!==b.nodeType?b:null},parents:function(a){return n.dir(a,"parentNode")},parentsUntil:function(a,b,c){return n.dir(a,"parentNode",c)},next:function(a){return D(a,"nextSibling")},prev:function(a){return D(a,"previousSibling")},nextAll:function(a){return n.dir(a,"nextSibling")},prevAll:function(a){return n.dir(a,"previousSibling")},nextUntil:function(a,b,c){return n.dir(a,"nextSibling",c)},prevUntil:function(a,b,c){return n.dir(a,"previousSibling",c)},siblings:function(a){return n.sibling((a.parentNode||{}).firstChild,a)},children:function(a){return n.sibling(a.firstChild)},contents:function(a){return a.contentDocument||n.merge([],a.childNodes)}},function(a,b){n.fn[a]=function(c,d){var e=n.map(this,b,c);return"Until"!==a.slice(-5)&&(d=c),d&&"string"==typeof d&&(e=n.filter(d,e)),this.length>1&&(C[a]||n.unique(e),B.test(a)&&e.reverse()),this.pushStack(e)}});var E=/\S+/g,F={};function G(a){var b=F[a]={};return n.each(a.match(E)||[],function(a,c){b[c]=!0}),b}n.Callbacks=function(a){a="string"==typeof a?F[a]||G(a):n.extend({},a);var b,c,d,e,f,g,h=[],i=!a.once&&[],j=function(l){for(b=a.memory&&l,c=!0,g=e||0,e=0,f=h.length,d=!0;h&&f>g;g++)if(h[g].apply(l[0],l[1])===!1&&a.stopOnFalse){b=!1;break}d=!1,h&&(i?i.length&&j(i.shift()):b?h=[]:k.disable())},k={add:function(){if(h){var c=h.length;!function g(b){n.each(b,function(b,c){var d=n.type(c);"function"===d?a.unique&&k.has(c)||h.push(c):c&&c.length&&"string"!==d&&g(c)})}(arguments),d?f=h.length:b&&(e=c,j(b))}return this},remove:function(){return h&&n.each(arguments,function(a,b){var c;while((c=n.inArray(b,h,c))>-1)h.splice(c,1),d&&(f>=c&&f--,g>=c&&g--)}),this},has:function(a){return a?n.inArray(a,h)>-1:!(!h||!h.length)},empty:function(){return h=[],f=0,this},disable:function(){return h=i=b=void 0,this},disabled:function(){return!h},lock:function(){return i=void 0,b||k.disable(),this},locked:function(){return!i},fireWith:function(a,b){return!h||c&&!i||(b=b||[],b=[a,b.slice?b.slice():b],d?i.push(b):j(b)),this},fire:function(){return k.fireWith(this,arguments),this},fired:function(){return!!c}};return k},n.extend({Deferred:function(a){var b=[["resolve","done",n.Callbacks("once memory"),"resolved"],["reject","fail",n.Callbacks("once memory"),"rejected"],["notify","progress",n.Callbacks("memory")]],c="pending",d={state:function(){return c},always:function(){return e.done(arguments).fail(arguments),this},then:function(){var a=arguments;return n.Deferred(function(c){n.each(b,function(b,f){var g=n.isFunction(a[b])&&a[b];e[f[1]](function(){var a=g&&g.apply(this,arguments);a&&n.isFunction(a.promise)?a.promise().done(c.resolve).fail(c.reject).progress(c.notify):c[f[0]+"With"](this===d?c.promise():this,g?[a]:arguments)})}),a=null}).promise()},promise:function(a){return null!=a?n.extend(a,d):d}},e={};return d.pipe=d.then,n.each(b,function(a,f){var g=f[2],h=f[3];d[f[1]]=g.add,h&&g.add(function(){c=h},b[1^a][2].disable,b[2][2].lock),e[f[0]]=function(){return e[f[0]+"With"](this===e?d:this,arguments),this},e[f[0]+"With"]=g.fireWith}),d.promise(e),a&&a.call(e,e),e},when:function(a){var b=0,c=d.call(arguments),e=c.length,f=1!==e||a&&n.isFunction(a.promise)?e:0,g=1===f?a:n.Deferred(),h=function(a,b,c){return function(e){b[a]=this,c[a]=arguments.length>1?d.call(arguments):e,c===i?g.notifyWith(b,c):--f||g.resolveWith(b,c)}},i,j,k;if(e>1)for(i=new Array(e),j=new Array(e),k=new Array(e);e>b;b++)c[b]&&n.isFunction(c[b].promise)?c[b].promise().done(h(b,k,c)).fail(g.reject).progress(h(b,j,i)):--f;return f||g.resolveWith(k,c),g.promise()}});var H;n.fn.ready=function(a){return n.ready.promise().done(a),this},n.extend({isReady:!1,readyWait:1,holdReady:function(a){a?n.readyWait++:n.ready(!0)},ready:function(a){(a===!0?--n.readyWait:n.isReady)||(n.isReady=!0,a!==!0&&--n.readyWait>0||(H.resolveWith(l,[n]),n.fn.triggerHandler&&(n(l).triggerHandler("ready"),n(l).off("ready"))))}});function I(){l.removeEventListener("DOMContentLoaded",I,!1),a.removeEventListener("load",I,!1),n.ready()}n.ready.promise=function(b){return H||(H=n.Deferred(),"complete"===l.readyState?setTimeout(n.ready):(l.addEventListener("DOMContentLoaded",I,!1),a.addEventListener("load",I,!1))),H.promise(b)},n.ready.promise();var J=n.access=function(a,b,c,d,e,f,g){var h=0,i=a.length,j=null==c;if("object"===n.type(c)){e=!0;for(h in c)n.access(a,b,h,c[h],!0,f,g)}else if(void 0!==d&&(e=!0,n.isFunction(d)||(g=!0),j&&(g?(b.call(a,d),b=null):(j=b,b=function(a,b,c){return j.call(n(a),c)})),b))for(;i>h;h++)b(a[h],c,g?d:d.call(a[h],h,b(a[h],c)));return e?a:j?b.call(a):i?b(a[0],c):f};n.acceptData=function(a){return 1===a.nodeType||9===a.nodeType||!+a.nodeType};function K(){Object.defineProperty(this.cache={},0,{get:function(){return{}}}),this.expando=n.expando+K.uid++}K.uid=1,K.accepts=n.acceptData,K.prototype={key:function(a){if(!K.accepts(a))return 0;var b={},c=a[this.expando];if(!c){c=K.uid++;try{b[this.expando]={value:c},Object.defineProperties(a,b)}catch(d){b[this.expando]=c,n.extend(a,b)}}return this.cache[c]||(this.cache[c]={}),c},set:function(a,b,c){var d,e=this.key(a),f=this.cache[e];if("string"==typeof b)f[b]=c;else if(n.isEmptyObject(f))n.extend(this.cache[e],b);else for(d in b)f[d]=b[d];return f},get:function(a,b){var c=this.cache[this.key(a)];return void 0===b?c:c[b]},access:function(a,b,c){var d;return void 0===b||b&&"string"==typeof b&&void 0===c?(d=this.get(a,b),void 0!==d?d:this.get(a,n.camelCase(b))):(this.set(a,b,c),void 0!==c?c:b)},remove:function(a,b){var c,d,e,f=this.key(a),g=this.cache[f];if(void 0===b)this.cache[f]={};else{n.isArray(b)?d=b.concat(b.map(n.camelCase)):(e=n.camelCase(b),b in g?d=[b,e]:(d=e,d=d in g?[d]:d.match(E)||[])),c=d.length;while(c--)delete g[d[c]]}},hasData:function(a){return!n.isEmptyObject(this.cache[a[this.expando]]||{})},discard:function(a){a[this.expando]&&delete this.cache[a[this.expando]]}};var L=new K,M=new K,N=/^(?:\{[\w\W]*\}|\[[\w\W]*\])$/,O=/([A-Z])/g;function P(a,b,c){var d;if(void 0===c&&1===a.nodeType)if(d="data-"+b.replace(O,"-$1").toLowerCase(),c=a.getAttribute(d),"string"==typeof c){try{c="true"===c?!0:"false"===c?!1:"null"===c?null:+c+""===c?+c:N.test(c)?n.parseJSON(c):c}catch(e){}M.set(a,b,c)}else c=void 0;return c}n.extend({hasData:function(a){return M.hasData(a)||L.hasData(a)},data:function(a,b,c){
+return M.access(a,b,c)},removeData:function(a,b){M.remove(a,b)},_data:function(a,b,c){return L.access(a,b,c)},_removeData:function(a,b){L.remove(a,b)}}),n.fn.extend({data:function(a,b){var c,d,e,f=this[0],g=f&&f.attributes;if(void 0===a){if(this.length&&(e=M.get(f),1===f.nodeType&&!L.get(f,"hasDataAttrs"))){c=g.length;while(c--)g[c]&&(d=g[c].name,0===d.indexOf("data-")&&(d=n.camelCase(d.slice(5)),P(f,d,e[d])));L.set(f,"hasDataAttrs",!0)}return e}return"object"==typeof a?this.each(function(){M.set(this,a)}):J(this,function(b){var c,d=n.camelCase(a);if(f&&void 0===b){if(c=M.get(f,a),void 0!==c)return c;if(c=M.get(f,d),void 0!==c)return c;if(c=P(f,d,void 0),void 0!==c)return c}else this.each(function(){var c=M.get(this,d);M.set(this,d,b),-1!==a.indexOf("-")&&void 0!==c&&M.set(this,a,b)})},null,b,arguments.length>1,null,!0)},removeData:function(a){return this.each(function(){M.remove(this,a)})}}),n.extend({queue:function(a,b,c){var d;return a?(b=(b||"fx")+"queue",d=L.get(a,b),c&&(!d||n.isArray(c)?d=L.access(a,b,n.makeArray(c)):d.push(c)),d||[]):void 0},dequeue:function(a,b){b=b||"fx";var c=n.queue(a,b),d=c.length,e=c.shift(),f=n._queueHooks(a,b),g=function(){n.dequeue(a,b)};"inprogress"===e&&(e=c.shift(),d--),e&&("fx"===b&&c.unshift("inprogress"),delete f.stop,e.call(a,g,f)),!d&&f&&f.empty.fire()},_queueHooks:function(a,b){var c=b+"queueHooks";return L.get(a,c)||L.access(a,c,{empty:n.Callbacks("once memory").add(function(){L.remove(a,[b+"queue",c])})})}}),n.fn.extend({queue:function(a,b){var c=2;return"string"!=typeof a&&(b=a,a="fx",c--),arguments.lengthx",k.noCloneChecked=!!b.cloneNode(!0).lastChild.defaultValue}();var U="undefined";k.focusinBubbles="onfocusin"in a;var V=/^key/,W=/^(?:mouse|pointer|contextmenu)|click/,X=/^(?:focusinfocus|focusoutblur)$/,Y=/^([^.]*)(?:\.(.+)|)$/;function Z(){return!0}function $(){return!1}function _(){try{return l.activeElement}catch(a){}}n.event={global:{},add:function(a,b,c,d,e){var f,g,h,i,j,k,l,m,o,p,q,r=L.get(a);if(r){c.handler&&(f=c,c=f.handler,e=f.selector),c.guid||(c.guid=n.guid++),(i=r.events)||(i=r.events={}),(g=r.handle)||(g=r.handle=function(b){return typeof n!==U&&n.event.triggered!==b.type?n.event.dispatch.apply(a,arguments):void 0}),b=(b||"").match(E)||[""],j=b.length;while(j--)h=Y.exec(b[j])||[],o=q=h[1],p=(h[2]||"").split(".").sort(),o&&(l=n.event.special[o]||{},o=(e?l.delegateType:l.bindType)||o,l=n.event.special[o]||{},k=n.extend({type:o,origType:q,data:d,handler:c,guid:c.guid,selector:e,needsContext:e&&n.expr.match.needsContext.test(e),namespace:p.join(".")},f),(m=i[o])||(m=i[o]=[],m.delegateCount=0,l.setup&&l.setup.call(a,d,p,g)!==!1||a.addEventListener&&a.addEventListener(o,g,!1)),l.add&&(l.add.call(a,k),k.handler.guid||(k.handler.guid=c.guid)),e?m.splice(m.delegateCount++,0,k):m.push(k),n.event.global[o]=!0)}},remove:function(a,b,c,d,e){var f,g,h,i,j,k,l,m,o,p,q,r=L.hasData(a)&&L.get(a);if(r&&(i=r.events)){b=(b||"").match(E)||[""],j=b.length;while(j--)if(h=Y.exec(b[j])||[],o=q=h[1],p=(h[2]||"").split(".").sort(),o){l=n.event.special[o]||{},o=(d?l.delegateType:l.bindType)||o,m=i[o]||[],h=h[2]&&new RegExp("(^|\\.)"+p.join("\\.(?:.*\\.|)")+"(\\.|$)"),g=f=m.length;while(f--)k=m[f],!e&&q!==k.origType||c&&c.guid!==k.guid||h&&!h.test(k.namespace)||d&&d!==k.selector&&("**"!==d||!k.selector)||(m.splice(f,1),k.selector&&m.delegateCount--,l.remove&&l.remove.call(a,k));g&&!m.length&&(l.teardown&&l.teardown.call(a,p,r.handle)!==!1||n.removeEvent(a,o,r.handle),delete i[o])}else for(o in i)n.event.remove(a,o+b[j],c,d,!0);n.isEmptyObject(i)&&(delete r.handle,L.remove(a,"events"))}},trigger:function(b,c,d,e){var f,g,h,i,k,m,o,p=[d||l],q=j.call(b,"type")?b.type:b,r=j.call(b,"namespace")?b.namespace.split("."):[];if(g=h=d=d||l,3!==d.nodeType&&8!==d.nodeType&&!X.test(q+n.event.triggered)&&(q.indexOf(".")>=0&&(r=q.split("."),q=r.shift(),r.sort()),k=q.indexOf(":")<0&&"on"+q,b=b[n.expando]?b:new n.Event(q,"object"==typeof b&&b),b.isTrigger=e?2:3,b.namespace=r.join("."),b.namespace_re=b.namespace?new RegExp("(^|\\.)"+r.join("\\.(?:.*\\.|)")+"(\\.|$)"):null,b.result=void 0,b.target||(b.target=d),c=null==c?[b]:n.makeArray(c,[b]),o=n.event.special[q]||{},e||!o.trigger||o.trigger.apply(d,c)!==!1)){if(!e&&!o.noBubble&&!n.isWindow(d)){for(i=o.delegateType||q,X.test(i+q)||(g=g.parentNode);g;g=g.parentNode)p.push(g),h=g;h===(d.ownerDocument||l)&&p.push(h.defaultView||h.parentWindow||a)}f=0;while((g=p[f++])&&!b.isPropagationStopped())b.type=f>1?i:o.bindType||q,m=(L.get(g,"events")||{})[b.type]&&L.get(g,"handle"),m&&m.apply(g,c),m=k&&g[k],m&&m.apply&&n.acceptData(g)&&(b.result=m.apply(g,c),b.result===!1&&b.preventDefault());return b.type=q,e||b.isDefaultPrevented()||o._default&&o._default.apply(p.pop(),c)!==!1||!n.acceptData(d)||k&&n.isFunction(d[q])&&!n.isWindow(d)&&(h=d[k],h&&(d[k]=null),n.event.triggered=q,d[q](),n.event.triggered=void 0,h&&(d[k]=h)),b.result}},dispatch:function(a){a=n.event.fix(a);var b,c,e,f,g,h=[],i=d.call(arguments),j=(L.get(this,"events")||{})[a.type]||[],k=n.event.special[a.type]||{};if(i[0]=a,a.delegateTarget=this,!k.preDispatch||k.preDispatch.call(this,a)!==!1){h=n.event.handlers.call(this,a,j),b=0;while((f=h[b++])&&!a.isPropagationStopped()){a.currentTarget=f.elem,c=0;while((g=f.handlers[c++])&&!a.isImmediatePropagationStopped())(!a.namespace_re||a.namespace_re.test(g.namespace))&&(a.handleObj=g,a.data=g.data,e=((n.event.special[g.origType]||{}).handle||g.handler).apply(f.elem,i),void 0!==e&&(a.result=e)===!1&&(a.preventDefault(),a.stopPropagation()))}return k.postDispatch&&k.postDispatch.call(this,a),a.result}},handlers:function(a,b){var c,d,e,f,g=[],h=b.delegateCount,i=a.target;if(h&&i.nodeType&&(!a.button||"click"!==a.type))for(;i!==this;i=i.parentNode||this)if(i.disabled!==!0||"click"!==a.type){for(d=[],c=0;h>c;c++)f=b[c],e=f.selector+" ",void 0===d[e]&&(d[e]=f.needsContext?n(e,this).index(i)>=0:n.find(e,this,null,[i]).length),d[e]&&d.push(f);d.length&&g.push({elem:i,handlers:d})}return h]*)\/>/gi,ba=/<([\w:]+)/,ca=/<|?\w+;/,da=/<(?:script|style|link)/i,ea=/checked\s*(?:[^=]|=\s*.checked.)/i,fa=/^$|\/(?:java|ecma)script/i,ga=/^true\/(.*)/,ha=/^\s*\s*$/g,ia={option:[1,""],thead:[1,""],col:[2,""],tr:[2,""],td:[3,""],_default:[0,"",""]};ia.optgroup=ia.option,ia.tbody=ia.tfoot=ia.colgroup=ia.caption=ia.thead,ia.th=ia.td;function ja(a,b){return n.nodeName(a,"table")&&n.nodeName(11!==b.nodeType?b:b.firstChild,"tr")?a.getElementsByTagName("tbody")[0]||a.appendChild(a.ownerDocument.createElement("tbody")):a}function ka(a){return a.type=(null!==a.getAttribute("type"))+"/"+a.type,a}function la(a){var b=ga.exec(a.type);return b?a.type=b[1]:a.removeAttribute("type"),a}function ma(a,b){for(var c=0,d=a.length;d>c;c++)L.set(a[c],"globalEval",!b||L.get(b[c],"globalEval"))}function na(a,b){var c,d,e,f,g,h,i,j;if(1===b.nodeType){if(L.hasData(a)&&(f=L.access(a),g=L.set(b,f),j=f.events)){delete g.handle,g.events={};for(e in j)for(c=0,d=j[e].length;d>c;c++)n.event.add(b,e,j[e][c])}M.hasData(a)&&(h=M.access(a),i=n.extend({},h),M.set(b,i))}}function oa(a,b){var c=a.getElementsByTagName?a.getElementsByTagName(b||"*"):a.querySelectorAll?a.querySelectorAll(b||"*"):[];return void 0===b||b&&n.nodeName(a,b)?n.merge([a],c):c}function pa(a,b){var c=b.nodeName.toLowerCase();"input"===c&&T.test(a.type)?b.checked=a.checked:("input"===c||"textarea"===c)&&(b.defaultValue=a.defaultValue)}n.extend({clone:function(a,b,c){var d,e,f,g,h=a.cloneNode(!0),i=n.contains(a.ownerDocument,a);if(!(k.noCloneChecked||1!==a.nodeType&&11!==a.nodeType||n.isXMLDoc(a)))for(g=oa(h),f=oa(a),d=0,e=f.length;e>d;d++)pa(f[d],g[d]);if(b)if(c)for(f=f||oa(a),g=g||oa(h),d=0,e=f.length;e>d;d++)na(f[d],g[d]);else na(a,h);return g=oa(h,"script"),g.length>0&&ma(g,!i&&oa(a,"script")),h},buildFragment:function(a,b,c,d){for(var e,f,g,h,i,j,k=b.createDocumentFragment(),l=[],m=0,o=a.length;o>m;m++)if(e=a[m],e||0===e)if("object"===n.type(e))n.merge(l,e.nodeType?[e]:e);else if(ca.test(e)){f=f||k.appendChild(b.createElement("div")),g=(ba.exec(e)||["",""])[1].toLowerCase(),h=ia[g]||ia._default,f.innerHTML=h[1]+e.replace(aa,"<$1>$2>")+h[2],j=h[0];while(j--)f=f.lastChild;n.merge(l,f.childNodes),f=k.firstChild,f.textContent=""}else l.push(b.createTextNode(e));k.textContent="",m=0;while(e=l[m++])if((!d||-1===n.inArray(e,d))&&(i=n.contains(e.ownerDocument,e),f=oa(k.appendChild(e),"script"),i&&ma(f),c)){j=0;while(e=f[j++])fa.test(e.type||"")&&c.push(e)}return k},cleanData:function(a){for(var b,c,d,e,f=n.event.special,g=0;void 0!==(c=a[g]);g++){if(n.acceptData(c)&&(e=c[L.expando],e&&(b=L.cache[e]))){if(b.events)for(d in b.events)f[d]?n.event.remove(c,d):n.removeEvent(c,d,b.handle);L.cache[e]&&delete L.cache[e]}delete M.cache[c[M.expando]]}}}),n.fn.extend({text:function(a){return J(this,function(a){return void 0===a?n.text(this):this.empty().each(function(){(1===this.nodeType||11===this.nodeType||9===this.nodeType)&&(this.textContent=a)})},null,a,arguments.length)},append:function(){return this.domManip(arguments,function(a){if(1===this.nodeType||11===this.nodeType||9===this.nodeType){var b=ja(this,a);b.appendChild(a)}})},prepend:function(){return this.domManip(arguments,function(a){if(1===this.nodeType||11===this.nodeType||9===this.nodeType){var b=ja(this,a);b.insertBefore(a,b.firstChild)}})},before:function(){return this.domManip(arguments,function(a){this.parentNode&&this.parentNode.insertBefore(a,this)})},after:function(){return this.domManip(arguments,function(a){this.parentNode&&this.parentNode.insertBefore(a,this.nextSibling)})},remove:function(a,b){for(var c,d=a?n.filter(a,this):this,e=0;null!=(c=d[e]);e++)b||1!==c.nodeType||n.cleanData(oa(c)),c.parentNode&&(b&&n.contains(c.ownerDocument,c)&&ma(oa(c,"script")),c.parentNode.removeChild(c));return this},empty:function(){for(var a,b=0;null!=(a=this[b]);b++)1===a.nodeType&&(n.cleanData(oa(a,!1)),a.textContent="");return this},clone:function(a,b){return a=null==a?!1:a,b=null==b?a:b,this.map(function(){return n.clone(this,a,b)})},html:function(a){return J(this,function(a){var b=this[0]||{},c=0,d=this.length;if(void 0===a&&1===b.nodeType)return b.innerHTML;if("string"==typeof a&&!da.test(a)&&!ia[(ba.exec(a)||["",""])[1].toLowerCase()]){a=a.replace(aa,"<$1>$2>");try{for(;d>c;c++)b=this[c]||{},1===b.nodeType&&(n.cleanData(oa(b,!1)),b.innerHTML=a);b=0}catch(e){}}b&&this.empty().append(a)},null,a,arguments.length)},replaceWith:function(){var a=arguments[0];return this.domManip(arguments,function(b){a=this.parentNode,n.cleanData(oa(this)),a&&a.replaceChild(b,this)}),a&&(a.length||a.nodeType)?this:this.remove()},detach:function(a){return this.remove(a,!0)},domManip:function(a,b){a=e.apply([],a);var c,d,f,g,h,i,j=0,l=this.length,m=this,o=l-1,p=a[0],q=n.isFunction(p);if(q||l>1&&"string"==typeof p&&!k.checkClone&&ea.test(p))return this.each(function(c){var d=m.eq(c);q&&(a[0]=p.call(this,c,d.html())),d.domManip(a,b)});if(l&&(c=n.buildFragment(a,this[0].ownerDocument,!1,this),d=c.firstChild,1===c.childNodes.length&&(c=d),d)){for(f=n.map(oa(c,"script"),ka),g=f.length;l>j;j++)h=c,j!==o&&(h=n.clone(h,!0,!0),g&&n.merge(f,oa(h,"script"))),b.call(this[j],h,j);if(g)for(i=f[f.length-1].ownerDocument,n.map(f,la),j=0;g>j;j++)h=f[j],fa.test(h.type||"")&&!L.access(h,"globalEval")&&n.contains(i,h)&&(h.src?n._evalUrl&&n._evalUrl(h.src):n.globalEval(h.textContent.replace(ha,"")))}return this}}),n.each({appendTo:"append",prependTo:"prepend",insertBefore:"before",insertAfter:"after",replaceAll:"replaceWith"},function(a,b){n.fn[a]=function(a){for(var c,d=[],e=n(a),g=e.length-1,h=0;g>=h;h++)c=h===g?this:this.clone(!0),n(e[h])[b](c),f.apply(d,c.get());return this.pushStack(d)}});var qa,ra={};function sa(b,c){var d,e=n(c.createElement(b)).appendTo(c.body),f=a.getDefaultComputedStyle&&(d=a.getDefaultComputedStyle(e[0]))?d.display:n.css(e[0],"display");return e.detach(),f}function ta(a){var b=l,c=ra[a];return c||(c=sa(a,b),"none"!==c&&c||(qa=(qa||n("")).appendTo(b.documentElement),b=qa[0].contentDocument,b.write(),b.close(),c=sa(a,b),qa.detach()),ra[a]=c),c}var ua=/^margin/,va=new RegExp("^("+Q+")(?!px)[a-z%]+$","i"),wa=function(b){return b.ownerDocument.defaultView.opener?b.ownerDocument.defaultView.getComputedStyle(b,null):a.getComputedStyle(b,null)};function xa(a,b,c){var d,e,f,g,h=a.style;return c=c||wa(a),c&&(g=c.getPropertyValue(b)||c[b]),c&&(""!==g||n.contains(a.ownerDocument,a)||(g=n.style(a,b)),va.test(g)&&ua.test(b)&&(d=h.width,e=h.minWidth,f=h.maxWidth,h.minWidth=h.maxWidth=h.width=g,g=c.width,h.width=d,h.minWidth=e,h.maxWidth=f)),void 0!==g?g+"":g}function ya(a,b){return{get:function(){return a()?void delete this.get:(this.get=b).apply(this,arguments)}}}!function(){var b,c,d=l.documentElement,e=l.createElement("div"),f=l.createElement("div");if(f.style){f.style.backgroundClip="content-box",f.cloneNode(!0).style.backgroundClip="",k.clearCloneStyle="content-box"===f.style.backgroundClip,e.style.cssText="border:0;width:0;height:0;top:0;left:-9999px;margin-top:1px;position:absolute",e.appendChild(f);function g(){f.style.cssText="-webkit-box-sizing:border-box;-moz-box-sizing:border-box;box-sizing:border-box;display:block;margin-top:1%;top:1%;border:1px;padding:1px;width:4px;position:absolute",f.innerHTML="",d.appendChild(e);var g=a.getComputedStyle(f,null);b="1%"!==g.top,c="4px"===g.width,d.removeChild(e)}a.getComputedStyle&&n.extend(k,{pixelPosition:function(){return g(),b},boxSizingReliable:function(){return null==c&&g(),c},reliableMarginRight:function(){var b,c=f.appendChild(l.createElement("div"));return c.style.cssText=f.style.cssText="-webkit-box-sizing:content-box;-moz-box-sizing:content-box;box-sizing:content-box;display:block;margin:0;border:0;padding:0",c.style.marginRight=c.style.width="0",f.style.width="1px",d.appendChild(e),b=!parseFloat(a.getComputedStyle(c,null).marginRight),d.removeChild(e),f.removeChild(c),b}})}}(),n.swap=function(a,b,c,d){var e,f,g={};for(f in b)g[f]=a.style[f],a.style[f]=b[f];e=c.apply(a,d||[]);for(f in b)a.style[f]=g[f];return e};var za=/^(none|table(?!-c[ea]).+)/,Aa=new RegExp("^("+Q+")(.*)$","i"),Ba=new RegExp("^([+-])=("+Q+")","i"),Ca={position:"absolute",visibility:"hidden",display:"block"},Da={letterSpacing:"0",fontWeight:"400"},Ea=["Webkit","O","Moz","ms"];function Fa(a,b){if(b in a)return b;var c=b[0].toUpperCase()+b.slice(1),d=b,e=Ea.length;while(e--)if(b=Ea[e]+c,b in a)return b;return d}function Ga(a,b,c){var d=Aa.exec(b);return d?Math.max(0,d[1]-(c||0))+(d[2]||"px"):b}function Ha(a,b,c,d,e){for(var f=c===(d?"border":"content")?4:"width"===b?1:0,g=0;4>f;f+=2)"margin"===c&&(g+=n.css(a,c+R[f],!0,e)),d?("content"===c&&(g-=n.css(a,"padding"+R[f],!0,e)),"margin"!==c&&(g-=n.css(a,"border"+R[f]+"Width",!0,e))):(g+=n.css(a,"padding"+R[f],!0,e),"padding"!==c&&(g+=n.css(a,"border"+R[f]+"Width",!0,e)));return g}function Ia(a,b,c){var d=!0,e="width"===b?a.offsetWidth:a.offsetHeight,f=wa(a),g="border-box"===n.css(a,"boxSizing",!1,f);if(0>=e||null==e){if(e=xa(a,b,f),(0>e||null==e)&&(e=a.style[b]),va.test(e))return e;d=g&&(k.boxSizingReliable()||e===a.style[b]),e=parseFloat(e)||0}return e+Ha(a,b,c||(g?"border":"content"),d,f)+"px"}function Ja(a,b){for(var c,d,e,f=[],g=0,h=a.length;h>g;g++)d=a[g],d.style&&(f[g]=L.get(d,"olddisplay"),c=d.style.display,b?(f[g]||"none"!==c||(d.style.display=""),""===d.style.display&&S(d)&&(f[g]=L.access(d,"olddisplay",ta(d.nodeName)))):(e=S(d),"none"===c&&e||L.set(d,"olddisplay",e?c:n.css(d,"display"))));for(g=0;h>g;g++)d=a[g],d.style&&(b&&"none"!==d.style.display&&""!==d.style.display||(d.style.display=b?f[g]||"":"none"));return a}n.extend({cssHooks:{opacity:{get:function(a,b){if(b){var c=xa(a,"opacity");return""===c?"1":c}}}},cssNumber:{columnCount:!0,fillOpacity:!0,flexGrow:!0,flexShrink:!0,fontWeight:!0,lineHeight:!0,opacity:!0,order:!0,orphans:!0,widows:!0,zIndex:!0,zoom:!0},cssProps:{"float":"cssFloat"},style:function(a,b,c,d){if(a&&3!==a.nodeType&&8!==a.nodeType&&a.style){var e,f,g,h=n.camelCase(b),i=a.style;return b=n.cssProps[h]||(n.cssProps[h]=Fa(i,h)),g=n.cssHooks[b]||n.cssHooks[h],void 0===c?g&&"get"in g&&void 0!==(e=g.get(a,!1,d))?e:i[b]:(f=typeof c,"string"===f&&(e=Ba.exec(c))&&(c=(e[1]+1)*e[2]+parseFloat(n.css(a,b)),f="number"),null!=c&&c===c&&("number"!==f||n.cssNumber[h]||(c+="px"),k.clearCloneStyle||""!==c||0!==b.indexOf("background")||(i[b]="inherit"),g&&"set"in g&&void 0===(c=g.set(a,c,d))||(i[b]=c)),void 0)}},css:function(a,b,c,d){var e,f,g,h=n.camelCase(b);return b=n.cssProps[h]||(n.cssProps[h]=Fa(a.style,h)),g=n.cssHooks[b]||n.cssHooks[h],g&&"get"in g&&(e=g.get(a,!0,c)),void 0===e&&(e=xa(a,b,d)),"normal"===e&&b in Da&&(e=Da[b]),""===c||c?(f=parseFloat(e),c===!0||n.isNumeric(f)?f||0:e):e}}),n.each(["height","width"],function(a,b){n.cssHooks[b]={get:function(a,c,d){return c?za.test(n.css(a,"display"))&&0===a.offsetWidth?n.swap(a,Ca,function(){return Ia(a,b,d)}):Ia(a,b,d):void 0},set:function(a,c,d){var e=d&&wa(a);return Ga(a,c,d?Ha(a,b,d,"border-box"===n.css(a,"boxSizing",!1,e),e):0)}}}),n.cssHooks.marginRight=ya(k.reliableMarginRight,function(a,b){return b?n.swap(a,{display:"inline-block"},xa,[a,"marginRight"]):void 0}),n.each({margin:"",padding:"",border:"Width"},function(a,b){n.cssHooks[a+b]={expand:function(c){for(var d=0,e={},f="string"==typeof c?c.split(" "):[c];4>d;d++)e[a+R[d]+b]=f[d]||f[d-2]||f[0];return e}},ua.test(a)||(n.cssHooks[a+b].set=Ga)}),n.fn.extend({css:function(a,b){return J(this,function(a,b,c){var d,e,f={},g=0;if(n.isArray(b)){for(d=wa(a),e=b.length;e>g;g++)f[b[g]]=n.css(a,b[g],!1,d);return f}return void 0!==c?n.style(a,b,c):n.css(a,b)},a,b,arguments.length>1)},show:function(){return Ja(this,!0)},hide:function(){return Ja(this)},toggle:function(a){return"boolean"==typeof a?a?this.show():this.hide():this.each(function(){S(this)?n(this).show():n(this).hide()})}});function Ka(a,b,c,d,e){return new Ka.prototype.init(a,b,c,d,e)}n.Tween=Ka,Ka.prototype={constructor:Ka,init:function(a,b,c,d,e,f){this.elem=a,this.prop=c,this.easing=e||"swing",this.options=b,this.start=this.now=this.cur(),this.end=d,this.unit=f||(n.cssNumber[c]?"":"px")},cur:function(){var a=Ka.propHooks[this.prop];return a&&a.get?a.get(this):Ka.propHooks._default.get(this)},run:function(a){var b,c=Ka.propHooks[this.prop];return this.options.duration?this.pos=b=n.easing[this.easing](a,this.options.duration*a,0,1,this.options.duration):this.pos=b=a,this.now=(this.end-this.start)*b+this.start,this.options.step&&this.options.step.call(this.elem,this.now,this),c&&c.set?c.set(this):Ka.propHooks._default.set(this),this}},Ka.prototype.init.prototype=Ka.prototype,Ka.propHooks={_default:{get:function(a){var b;return null==a.elem[a.prop]||a.elem.style&&null!=a.elem.style[a.prop]?(b=n.css(a.elem,a.prop,""),b&&"auto"!==b?b:0):a.elem[a.prop]},set:function(a){n.fx.step[a.prop]?n.fx.step[a.prop](a):a.elem.style&&(null!=a.elem.style[n.cssProps[a.prop]]||n.cssHooks[a.prop])?n.style(a.elem,a.prop,a.now+a.unit):a.elem[a.prop]=a.now}}},Ka.propHooks.scrollTop=Ka.propHooks.scrollLeft={set:function(a){a.elem.nodeType&&a.elem.parentNode&&(a.elem[a.prop]=a.now)}},n.easing={linear:function(a){return a},swing:function(a){return.5-Math.cos(a*Math.PI)/2}},n.fx=Ka.prototype.init,n.fx.step={};var La,Ma,Na=/^(?:toggle|show|hide)$/,Oa=new RegExp("^(?:([+-])=|)("+Q+")([a-z%]*)$","i"),Pa=/queueHooks$/,Qa=[Va],Ra={"*":[function(a,b){var c=this.createTween(a,b),d=c.cur(),e=Oa.exec(b),f=e&&e[3]||(n.cssNumber[a]?"":"px"),g=(n.cssNumber[a]||"px"!==f&&+d)&&Oa.exec(n.css(c.elem,a)),h=1,i=20;if(g&&g[3]!==f){f=f||g[3],e=e||[],g=+d||1;do h=h||".5",g/=h,n.style(c.elem,a,g+f);while(h!==(h=c.cur()/d)&&1!==h&&--i)}return e&&(g=c.start=+g||+d||0,c.unit=f,c.end=e[1]?g+(e[1]+1)*e[2]:+e[2]),c}]};function Sa(){return setTimeout(function(){La=void 0}),La=n.now()}function Ta(a,b){var c,d=0,e={height:a};for(b=b?1:0;4>d;d+=2-b)c=R[d],e["margin"+c]=e["padding"+c]=a;return b&&(e.opacity=e.width=a),e}function Ua(a,b,c){for(var d,e=(Ra[b]||[]).concat(Ra["*"]),f=0,g=e.length;g>f;f++)if(d=e[f].call(c,b,a))return d}function Va(a,b,c){var d,e,f,g,h,i,j,k,l=this,m={},o=a.style,p=a.nodeType&&S(a),q=L.get(a,"fxshow");c.queue||(h=n._queueHooks(a,"fx"),null==h.unqueued&&(h.unqueued=0,i=h.empty.fire,h.empty.fire=function(){h.unqueued||i()}),h.unqueued++,l.always(function(){l.always(function(){h.unqueued--,n.queue(a,"fx").length||h.empty.fire()})})),1===a.nodeType&&("height"in b||"width"in b)&&(c.overflow=[o.overflow,o.overflowX,o.overflowY],j=n.css(a,"display"),k="none"===j?L.get(a,"olddisplay")||ta(a.nodeName):j,"inline"===k&&"none"===n.css(a,"float")&&(o.display="inline-block")),c.overflow&&(o.overflow="hidden",l.always(function(){o.overflow=c.overflow[0],o.overflowX=c.overflow[1],o.overflowY=c.overflow[2]}));for(d in b)if(e=b[d],Na.exec(e)){if(delete b[d],f=f||"toggle"===e,e===(p?"hide":"show")){if("show"!==e||!q||void 0===q[d])continue;p=!0}m[d]=q&&q[d]||n.style(a,d)}else j=void 0;if(n.isEmptyObject(m))"inline"===("none"===j?ta(a.nodeName):j)&&(o.display=j);else{q?"hidden"in q&&(p=q.hidden):q=L.access(a,"fxshow",{}),f&&(q.hidden=!p),p?n(a).show():l.done(function(){n(a).hide()}),l.done(function(){var b;L.remove(a,"fxshow");for(b in m)n.style(a,b,m[b])});for(d in m)g=Ua(p?q[d]:0,d,l),d in q||(q[d]=g.start,p&&(g.end=g.start,g.start="width"===d||"height"===d?1:0))}}function Wa(a,b){var c,d,e,f,g;for(c in a)if(d=n.camelCase(c),e=b[d],f=a[c],n.isArray(f)&&(e=f[1],f=a[c]=f[0]),c!==d&&(a[d]=f,delete a[c]),g=n.cssHooks[d],g&&"expand"in g){f=g.expand(f),delete a[d];for(c in f)c in a||(a[c]=f[c],b[c]=e)}else b[d]=e}function Xa(a,b,c){var d,e,f=0,g=Qa.length,h=n.Deferred().always(function(){delete i.elem}),i=function(){if(e)return!1;for(var b=La||Sa(),c=Math.max(0,j.startTime+j.duration-b),d=c/j.duration||0,f=1-d,g=0,i=j.tweens.length;i>g;g++)j.tweens[g].run(f);return h.notifyWith(a,[j,f,c]),1>f&&i?c:(h.resolveWith(a,[j]),!1)},j=h.promise({elem:a,props:n.extend({},b),opts:n.extend(!0,{specialEasing:{}},c),originalProperties:b,originalOptions:c,startTime:La||Sa(),duration:c.duration,tweens:[],createTween:function(b,c){var d=n.Tween(a,j.opts,b,c,j.opts.specialEasing[b]||j.opts.easing);return j.tweens.push(d),d},stop:function(b){var c=0,d=b?j.tweens.length:0;if(e)return this;for(e=!0;d>c;c++)j.tweens[c].run(1);return b?h.resolveWith(a,[j,b]):h.rejectWith(a,[j,b]),this}}),k=j.props;for(Wa(k,j.opts.specialEasing);g>f;f++)if(d=Qa[f].call(j,a,k,j.opts))return d;return n.map(k,Ua,j),n.isFunction(j.opts.start)&&j.opts.start.call(a,j),n.fx.timer(n.extend(i,{elem:a,anim:j,queue:j.opts.queue})),j.progress(j.opts.progress).done(j.opts.done,j.opts.complete).fail(j.opts.fail).always(j.opts.always)}n.Animation=n.extend(Xa,{tweener:function(a,b){n.isFunction(a)?(b=a,a=["*"]):a=a.split(" ");for(var c,d=0,e=a.length;e>d;d++)c=a[d],Ra[c]=Ra[c]||[],Ra[c].unshift(b)},prefilter:function(a,b){b?Qa.unshift(a):Qa.push(a)}}),n.speed=function(a,b,c){var d=a&&"object"==typeof a?n.extend({},a):{complete:c||!c&&b||n.isFunction(a)&&a,duration:a,easing:c&&b||b&&!n.isFunction(b)&&b};return d.duration=n.fx.off?0:"number"==typeof d.duration?d.duration:d.duration in n.fx.speeds?n.fx.speeds[d.duration]:n.fx.speeds._default,(null==d.queue||d.queue===!0)&&(d.queue="fx"),d.old=d.complete,d.complete=function(){n.isFunction(d.old)&&d.old.call(this),d.queue&&n.dequeue(this,d.queue)},d},n.fn.extend({fadeTo:function(a,b,c,d){return this.filter(S).css("opacity",0).show().end().animate({opacity:b},a,c,d)},animate:function(a,b,c,d){var e=n.isEmptyObject(a),f=n.speed(b,c,d),g=function(){var b=Xa(this,n.extend({},a),f);(e||L.get(this,"finish"))&&b.stop(!0)};return g.finish=g,e||f.queue===!1?this.each(g):this.queue(f.queue,g)},stop:function(a,b,c){var d=function(a){var b=a.stop;delete a.stop,b(c)};return"string"!=typeof a&&(c=b,b=a,a=void 0),b&&a!==!1&&this.queue(a||"fx",[]),this.each(function(){var b=!0,e=null!=a&&a+"queueHooks",f=n.timers,g=L.get(this);if(e)g[e]&&g[e].stop&&d(g[e]);else for(e in g)g[e]&&g[e].stop&&Pa.test(e)&&d(g[e]);for(e=f.length;e--;)f[e].elem!==this||null!=a&&f[e].queue!==a||(f[e].anim.stop(c),b=!1,f.splice(e,1));(b||!c)&&n.dequeue(this,a)})},finish:function(a){return a!==!1&&(a=a||"fx"),this.each(function(){var b,c=L.get(this),d=c[a+"queue"],e=c[a+"queueHooks"],f=n.timers,g=d?d.length:0;for(c.finish=!0,n.queue(this,a,[]),e&&e.stop&&e.stop.call(this,!0),b=f.length;b--;)f[b].elem===this&&f[b].queue===a&&(f[b].anim.stop(!0),f.splice(b,1));for(b=0;g>b;b++)d[b]&&d[b].finish&&d[b].finish.call(this);delete c.finish})}}),n.each(["toggle","show","hide"],function(a,b){var c=n.fn[b];n.fn[b]=function(a,d,e){return null==a||"boolean"==typeof a?c.apply(this,arguments):this.animate(Ta(b,!0),a,d,e)}}),n.each({slideDown:Ta("show"),slideUp:Ta("hide"),slideToggle:Ta("toggle"),fadeIn:{opacity:"show"},fadeOut:{opacity:"hide"},fadeToggle:{opacity:"toggle"}},function(a,b){n.fn[a]=function(a,c,d){return this.animate(b,a,c,d)}}),n.timers=[],n.fx.tick=function(){var a,b=0,c=n.timers;for(La=n.now();b1)},removeAttr:function(a){return this.each(function(){n.removeAttr(this,a)})}}),n.extend({attr:function(a,b,c){var d,e,f=a.nodeType;if(a&&3!==f&&8!==f&&2!==f)return typeof a.getAttribute===U?n.prop(a,b,c):(1===f&&n.isXMLDoc(a)||(b=b.toLowerCase(),d=n.attrHooks[b]||(n.expr.match.bool.test(b)?Za:Ya)),
+void 0===c?d&&"get"in d&&null!==(e=d.get(a,b))?e:(e=n.find.attr(a,b),null==e?void 0:e):null!==c?d&&"set"in d&&void 0!==(e=d.set(a,c,b))?e:(a.setAttribute(b,c+""),c):void n.removeAttr(a,b))},removeAttr:function(a,b){var c,d,e=0,f=b&&b.match(E);if(f&&1===a.nodeType)while(c=f[e++])d=n.propFix[c]||c,n.expr.match.bool.test(c)&&(a[d]=!1),a.removeAttribute(c)},attrHooks:{type:{set:function(a,b){if(!k.radioValue&&"radio"===b&&n.nodeName(a,"input")){var c=a.value;return a.setAttribute("type",b),c&&(a.value=c),b}}}}}),Za={set:function(a,b,c){return b===!1?n.removeAttr(a,c):a.setAttribute(c,c),c}},n.each(n.expr.match.bool.source.match(/\w+/g),function(a,b){var c=$a[b]||n.find.attr;$a[b]=function(a,b,d){var e,f;return d||(f=$a[b],$a[b]=e,e=null!=c(a,b,d)?b.toLowerCase():null,$a[b]=f),e}});var _a=/^(?:input|select|textarea|button)$/i;n.fn.extend({prop:function(a,b){return J(this,n.prop,a,b,arguments.length>1)},removeProp:function(a){return this.each(function(){delete this[n.propFix[a]||a]})}}),n.extend({propFix:{"for":"htmlFor","class":"className"},prop:function(a,b,c){var d,e,f,g=a.nodeType;if(a&&3!==g&&8!==g&&2!==g)return f=1!==g||!n.isXMLDoc(a),f&&(b=n.propFix[b]||b,e=n.propHooks[b]),void 0!==c?e&&"set"in e&&void 0!==(d=e.set(a,c,b))?d:a[b]=c:e&&"get"in e&&null!==(d=e.get(a,b))?d:a[b]},propHooks:{tabIndex:{get:function(a){return a.hasAttribute("tabindex")||_a.test(a.nodeName)||a.href?a.tabIndex:-1}}}}),k.optSelected||(n.propHooks.selected={get:function(a){var b=a.parentNode;return b&&b.parentNode&&b.parentNode.selectedIndex,null}}),n.each(["tabIndex","readOnly","maxLength","cellSpacing","cellPadding","rowSpan","colSpan","useMap","frameBorder","contentEditable"],function(){n.propFix[this.toLowerCase()]=this});var ab=/[\t\r\n\f]/g;n.fn.extend({addClass:function(a){var b,c,d,e,f,g,h="string"==typeof a&&a,i=0,j=this.length;if(n.isFunction(a))return this.each(function(b){n(this).addClass(a.call(this,b,this.className))});if(h)for(b=(a||"").match(E)||[];j>i;i++)if(c=this[i],d=1===c.nodeType&&(c.className?(" "+c.className+" ").replace(ab," "):" ")){f=0;while(e=b[f++])d.indexOf(" "+e+" ")<0&&(d+=e+" ");g=n.trim(d),c.className!==g&&(c.className=g)}return this},removeClass:function(a){var b,c,d,e,f,g,h=0===arguments.length||"string"==typeof a&&a,i=0,j=this.length;if(n.isFunction(a))return this.each(function(b){n(this).removeClass(a.call(this,b,this.className))});if(h)for(b=(a||"").match(E)||[];j>i;i++)if(c=this[i],d=1===c.nodeType&&(c.className?(" "+c.className+" ").replace(ab," "):"")){f=0;while(e=b[f++])while(d.indexOf(" "+e+" ")>=0)d=d.replace(" "+e+" "," ");g=a?n.trim(d):"",c.className!==g&&(c.className=g)}return this},toggleClass:function(a,b){var c=typeof a;return"boolean"==typeof b&&"string"===c?b?this.addClass(a):this.removeClass(a):this.each(n.isFunction(a)?function(c){n(this).toggleClass(a.call(this,c,this.className,b),b)}:function(){if("string"===c){var b,d=0,e=n(this),f=a.match(E)||[];while(b=f[d++])e.hasClass(b)?e.removeClass(b):e.addClass(b)}else(c===U||"boolean"===c)&&(this.className&&L.set(this,"__className__",this.className),this.className=this.className||a===!1?"":L.get(this,"__className__")||"")})},hasClass:function(a){for(var b=" "+a+" ",c=0,d=this.length;d>c;c++)if(1===this[c].nodeType&&(" "+this[c].className+" ").replace(ab," ").indexOf(b)>=0)return!0;return!1}});var bb=/\r/g;n.fn.extend({val:function(a){var b,c,d,e=this[0];{if(arguments.length)return d=n.isFunction(a),this.each(function(c){var e;1===this.nodeType&&(e=d?a.call(this,c,n(this).val()):a,null==e?e="":"number"==typeof e?e+="":n.isArray(e)&&(e=n.map(e,function(a){return null==a?"":a+""})),b=n.valHooks[this.type]||n.valHooks[this.nodeName.toLowerCase()],b&&"set"in b&&void 0!==b.set(this,e,"value")||(this.value=e))});if(e)return b=n.valHooks[e.type]||n.valHooks[e.nodeName.toLowerCase()],b&&"get"in b&&void 0!==(c=b.get(e,"value"))?c:(c=e.value,"string"==typeof c?c.replace(bb,""):null==c?"":c)}}}),n.extend({valHooks:{option:{get:function(a){var b=n.find.attr(a,"value");return null!=b?b:n.trim(n.text(a))}},select:{get:function(a){for(var b,c,d=a.options,e=a.selectedIndex,f="select-one"===a.type||0>e,g=f?null:[],h=f?e+1:d.length,i=0>e?h:f?e:0;h>i;i++)if(c=d[i],!(!c.selected&&i!==e||(k.optDisabled?c.disabled:null!==c.getAttribute("disabled"))||c.parentNode.disabled&&n.nodeName(c.parentNode,"optgroup"))){if(b=n(c).val(),f)return b;g.push(b)}return g},set:function(a,b){var c,d,e=a.options,f=n.makeArray(b),g=e.length;while(g--)d=e[g],(d.selected=n.inArray(d.value,f)>=0)&&(c=!0);return c||(a.selectedIndex=-1),f}}}}),n.each(["radio","checkbox"],function(){n.valHooks[this]={set:function(a,b){return n.isArray(b)?a.checked=n.inArray(n(a).val(),b)>=0:void 0}},k.checkOn||(n.valHooks[this].get=function(a){return null===a.getAttribute("value")?"on":a.value})}),n.each("blur focus focusin focusout load resize scroll unload click dblclick mousedown mouseup mousemove mouseover mouseout mouseenter mouseleave change select submit keydown keypress keyup error contextmenu".split(" "),function(a,b){n.fn[b]=function(a,c){return arguments.length>0?this.on(b,null,a,c):this.trigger(b)}}),n.fn.extend({hover:function(a,b){return this.mouseenter(a).mouseleave(b||a)},bind:function(a,b,c){return this.on(a,null,b,c)},unbind:function(a,b){return this.off(a,null,b)},delegate:function(a,b,c,d){return this.on(b,a,c,d)},undelegate:function(a,b,c){return 1===arguments.length?this.off(a,"**"):this.off(b,a||"**",c)}});var cb=n.now(),db=/\?/;n.parseJSON=function(a){return JSON.parse(a+"")},n.parseXML=function(a){var b,c;if(!a||"string"!=typeof a)return null;try{c=new DOMParser,b=c.parseFromString(a,"text/xml")}catch(d){b=void 0}return(!b||b.getElementsByTagName("parsererror").length)&&n.error("Invalid XML: "+a),b};var eb=/#.*$/,fb=/([?&])_=[^&]*/,gb=/^(.*?):[ \t]*([^\r\n]*)$/gm,hb=/^(?:about|app|app-storage|.+-extension|file|res|widget):$/,ib=/^(?:GET|HEAD)$/,jb=/^\/\//,kb=/^([\w.+-]+:)(?:\/\/(?:[^\/?#]*@|)([^\/?#:]*)(?::(\d+)|)|)/,lb={},mb={},nb="*/".concat("*"),ob=a.location.href,pb=kb.exec(ob.toLowerCase())||[];function qb(a){return function(b,c){"string"!=typeof b&&(c=b,b="*");var d,e=0,f=b.toLowerCase().match(E)||[];if(n.isFunction(c))while(d=f[e++])"+"===d[0]?(d=d.slice(1)||"*",(a[d]=a[d]||[]).unshift(c)):(a[d]=a[d]||[]).push(c)}}function rb(a,b,c,d){var e={},f=a===mb;function g(h){var i;return e[h]=!0,n.each(a[h]||[],function(a,h){var j=h(b,c,d);return"string"!=typeof j||f||e[j]?f?!(i=j):void 0:(b.dataTypes.unshift(j),g(j),!1)}),i}return g(b.dataTypes[0])||!e["*"]&&g("*")}function sb(a,b){var c,d,e=n.ajaxSettings.flatOptions||{};for(c in b)void 0!==b[c]&&((e[c]?a:d||(d={}))[c]=b[c]);return d&&n.extend(!0,a,d),a}function tb(a,b,c){var d,e,f,g,h=a.contents,i=a.dataTypes;while("*"===i[0])i.shift(),void 0===d&&(d=a.mimeType||b.getResponseHeader("Content-Type"));if(d)for(e in h)if(h[e]&&h[e].test(d)){i.unshift(e);break}if(i[0]in c)f=i[0];else{for(e in c){if(!i[0]||a.converters[e+" "+i[0]]){f=e;break}g||(g=e)}f=f||g}return f?(f!==i[0]&&i.unshift(f),c[f]):void 0}function ub(a,b,c,d){var e,f,g,h,i,j={},k=a.dataTypes.slice();if(k[1])for(g in a.converters)j[g.toLowerCase()]=a.converters[g];f=k.shift();while(f)if(a.responseFields[f]&&(c[a.responseFields[f]]=b),!i&&d&&a.dataFilter&&(b=a.dataFilter(b,a.dataType)),i=f,f=k.shift())if("*"===f)f=i;else if("*"!==i&&i!==f){if(g=j[i+" "+f]||j["* "+f],!g)for(e in j)if(h=e.split(" "),h[1]===f&&(g=j[i+" "+h[0]]||j["* "+h[0]])){g===!0?g=j[e]:j[e]!==!0&&(f=h[0],k.unshift(h[1]));break}if(g!==!0)if(g&&a["throws"])b=g(b);else try{b=g(b)}catch(l){return{state:"parsererror",error:g?l:"No conversion from "+i+" to "+f}}}return{state:"success",data:b}}n.extend({active:0,lastModified:{},etag:{},ajaxSettings:{url:ob,type:"GET",isLocal:hb.test(pb[1]),global:!0,processData:!0,async:!0,contentType:"application/x-www-form-urlencoded; charset=UTF-8",accepts:{"*":nb,text:"text/plain",html:"text/html",xml:"application/xml, text/xml",json:"application/json, text/javascript"},contents:{xml:/xml/,html:/html/,json:/json/},responseFields:{xml:"responseXML",text:"responseText",json:"responseJSON"},converters:{"* text":String,"text html":!0,"text json":n.parseJSON,"text xml":n.parseXML},flatOptions:{url:!0,context:!0}},ajaxSetup:function(a,b){return b?sb(sb(a,n.ajaxSettings),b):sb(n.ajaxSettings,a)},ajaxPrefilter:qb(lb),ajaxTransport:qb(mb),ajax:function(a,b){"object"==typeof a&&(b=a,a=void 0),b=b||{};var c,d,e,f,g,h,i,j,k=n.ajaxSetup({},b),l=k.context||k,m=k.context&&(l.nodeType||l.jquery)?n(l):n.event,o=n.Deferred(),p=n.Callbacks("once memory"),q=k.statusCode||{},r={},s={},t=0,u="canceled",v={readyState:0,getResponseHeader:function(a){var b;if(2===t){if(!f){f={};while(b=gb.exec(e))f[b[1].toLowerCase()]=b[2]}b=f[a.toLowerCase()]}return null==b?null:b},getAllResponseHeaders:function(){return 2===t?e:null},setRequestHeader:function(a,b){var c=a.toLowerCase();return t||(a=s[c]=s[c]||a,r[a]=b),this},overrideMimeType:function(a){return t||(k.mimeType=a),this},statusCode:function(a){var b;if(a)if(2>t)for(b in a)q[b]=[q[b],a[b]];else v.always(a[v.status]);return this},abort:function(a){var b=a||u;return c&&c.abort(b),x(0,b),this}};if(o.promise(v).complete=p.add,v.success=v.done,v.error=v.fail,k.url=((a||k.url||ob)+"").replace(eb,"").replace(jb,pb[1]+"//"),k.type=b.method||b.type||k.method||k.type,k.dataTypes=n.trim(k.dataType||"*").toLowerCase().match(E)||[""],null==k.crossDomain&&(h=kb.exec(k.url.toLowerCase()),k.crossDomain=!(!h||h[1]===pb[1]&&h[2]===pb[2]&&(h[3]||("http:"===h[1]?"80":"443"))===(pb[3]||("http:"===pb[1]?"80":"443")))),k.data&&k.processData&&"string"!=typeof k.data&&(k.data=n.param(k.data,k.traditional)),rb(lb,k,b,v),2===t)return v;i=n.event&&k.global,i&&0===n.active++&&n.event.trigger("ajaxStart"),k.type=k.type.toUpperCase(),k.hasContent=!ib.test(k.type),d=k.url,k.hasContent||(k.data&&(d=k.url+=(db.test(d)?"&":"?")+k.data,delete k.data),k.cache===!1&&(k.url=fb.test(d)?d.replace(fb,"$1_="+cb++):d+(db.test(d)?"&":"?")+"_="+cb++)),k.ifModified&&(n.lastModified[d]&&v.setRequestHeader("If-Modified-Since",n.lastModified[d]),n.etag[d]&&v.setRequestHeader("If-None-Match",n.etag[d])),(k.data&&k.hasContent&&k.contentType!==!1||b.contentType)&&v.setRequestHeader("Content-Type",k.contentType),v.setRequestHeader("Accept",k.dataTypes[0]&&k.accepts[k.dataTypes[0]]?k.accepts[k.dataTypes[0]]+("*"!==k.dataTypes[0]?", "+nb+"; q=0.01":""):k.accepts["*"]);for(j in k.headers)v.setRequestHeader(j,k.headers[j]);if(k.beforeSend&&(k.beforeSend.call(l,v,k)===!1||2===t))return v.abort();u="abort";for(j in{success:1,error:1,complete:1})v[j](k[j]);if(c=rb(mb,k,b,v)){v.readyState=1,i&&m.trigger("ajaxSend",[v,k]),k.async&&k.timeout>0&&(g=setTimeout(function(){v.abort("timeout")},k.timeout));try{t=1,c.send(r,x)}catch(w){if(!(2>t))throw w;x(-1,w)}}else x(-1,"No Transport");function x(a,b,f,h){var j,r,s,u,w,x=b;2!==t&&(t=2,g&&clearTimeout(g),c=void 0,e=h||"",v.readyState=a>0?4:0,j=a>=200&&300>a||304===a,f&&(u=tb(k,v,f)),u=ub(k,u,v,j),j?(k.ifModified&&(w=v.getResponseHeader("Last-Modified"),w&&(n.lastModified[d]=w),w=v.getResponseHeader("etag"),w&&(n.etag[d]=w)),204===a||"HEAD"===k.type?x="nocontent":304===a?x="notmodified":(x=u.state,r=u.data,s=u.error,j=!s)):(s=x,(a||!x)&&(x="error",0>a&&(a=0))),v.status=a,v.statusText=(b||x)+"",j?o.resolveWith(l,[r,x,v]):o.rejectWith(l,[v,x,s]),v.statusCode(q),q=void 0,i&&m.trigger(j?"ajaxSuccess":"ajaxError",[v,k,j?r:s]),p.fireWith(l,[v,x]),i&&(m.trigger("ajaxComplete",[v,k]),--n.active||n.event.trigger("ajaxStop")))}return v},getJSON:function(a,b,c){return n.get(a,b,c,"json")},getScript:function(a,b){return n.get(a,void 0,b,"script")}}),n.each(["get","post"],function(a,b){n[b]=function(a,c,d,e){return n.isFunction(c)&&(e=e||d,d=c,c=void 0),n.ajax({url:a,type:b,dataType:e,data:c,success:d})}}),n._evalUrl=function(a){return n.ajax({url:a,type:"GET",dataType:"script",async:!1,global:!1,"throws":!0})},n.fn.extend({wrapAll:function(a){var b;return n.isFunction(a)?this.each(function(b){n(this).wrapAll(a.call(this,b))}):(this[0]&&(b=n(a,this[0].ownerDocument).eq(0).clone(!0),this[0].parentNode&&b.insertBefore(this[0]),b.map(function(){var a=this;while(a.firstElementChild)a=a.firstElementChild;return a}).append(this)),this)},wrapInner:function(a){return this.each(n.isFunction(a)?function(b){n(this).wrapInner(a.call(this,b))}:function(){var b=n(this),c=b.contents();c.length?c.wrapAll(a):b.append(a)})},wrap:function(a){var b=n.isFunction(a);return this.each(function(c){n(this).wrapAll(b?a.call(this,c):a)})},unwrap:function(){return this.parent().each(function(){n.nodeName(this,"body")||n(this).replaceWith(this.childNodes)}).end()}}),n.expr.filters.hidden=function(a){return a.offsetWidth<=0&&a.offsetHeight<=0},n.expr.filters.visible=function(a){return!n.expr.filters.hidden(a)};var vb=/%20/g,wb=/\[\]$/,xb=/\r?\n/g,yb=/^(?:submit|button|image|reset|file)$/i,zb=/^(?:input|select|textarea|keygen)/i;function Ab(a,b,c,d){var e;if(n.isArray(b))n.each(b,function(b,e){c||wb.test(a)?d(a,e):Ab(a+"["+("object"==typeof e?b:"")+"]",e,c,d)});else if(c||"object"!==n.type(b))d(a,b);else for(e in b)Ab(a+"["+e+"]",b[e],c,d)}n.param=function(a,b){var c,d=[],e=function(a,b){b=n.isFunction(b)?b():null==b?"":b,d[d.length]=encodeURIComponent(a)+"="+encodeURIComponent(b)};if(void 0===b&&(b=n.ajaxSettings&&n.ajaxSettings.traditional),n.isArray(a)||a.jquery&&!n.isPlainObject(a))n.each(a,function(){e(this.name,this.value)});else for(c in a)Ab(c,a[c],b,e);return d.join("&").replace(vb,"+")},n.fn.extend({serialize:function(){return n.param(this.serializeArray())},serializeArray:function(){return this.map(function(){var a=n.prop(this,"elements");return a?n.makeArray(a):this}).filter(function(){var a=this.type;return this.name&&!n(this).is(":disabled")&&zb.test(this.nodeName)&&!yb.test(a)&&(this.checked||!T.test(a))}).map(function(a,b){var c=n(this).val();return null==c?null:n.isArray(c)?n.map(c,function(a){return{name:b.name,value:a.replace(xb,"\r\n")}}):{name:b.name,value:c.replace(xb,"\r\n")}}).get()}}),n.ajaxSettings.xhr=function(){try{return new XMLHttpRequest}catch(a){}};var Bb=0,Cb={},Db={0:200,1223:204},Eb=n.ajaxSettings.xhr();a.attachEvent&&a.attachEvent("onunload",function(){for(var a in Cb)Cb[a]()}),k.cors=!!Eb&&"withCredentials"in Eb,k.ajax=Eb=!!Eb,n.ajaxTransport(function(a){var b;return k.cors||Eb&&!a.crossDomain?{send:function(c,d){var e,f=a.xhr(),g=++Bb;if(f.open(a.type,a.url,a.async,a.username,a.password),a.xhrFields)for(e in a.xhrFields)f[e]=a.xhrFields[e];a.mimeType&&f.overrideMimeType&&f.overrideMimeType(a.mimeType),a.crossDomain||c["X-Requested-With"]||(c["X-Requested-With"]="XMLHttpRequest");for(e in c)f.setRequestHeader(e,c[e]);b=function(a){return function(){b&&(delete Cb[g],b=f.onload=f.onerror=null,"abort"===a?f.abort():"error"===a?d(f.status,f.statusText):d(Db[f.status]||f.status,f.statusText,"string"==typeof f.responseText?{text:f.responseText}:void 0,f.getAllResponseHeaders()))}},f.onload=b(),f.onerror=b("error"),b=Cb[g]=b("abort");try{f.send(a.hasContent&&a.data||null)}catch(h){if(b)throw h}},abort:function(){b&&b()}}:void 0}),n.ajaxSetup({accepts:{script:"text/javascript, application/javascript, application/ecmascript, application/x-ecmascript"},contents:{script:/(?:java|ecma)script/},converters:{"text script":function(a){return n.globalEval(a),a}}}),n.ajaxPrefilter("script",function(a){void 0===a.cache&&(a.cache=!1),a.crossDomain&&(a.type="GET")}),n.ajaxTransport("script",function(a){if(a.crossDomain){var b,c;return{send:function(d,e){b=n("
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ CanonnED3D - Maps
+
+
+
+
+
+
+
+
+
+
+
+

+
+
+
+
+
+
+
+
+
+
+