diff --git a/contributing/content-markup-reference.md b/contributing/content-markup-reference.md
index 0298123710ff..ed376df1a3e3 100644
--- a/contributing/content-markup-reference.md
+++ b/contributing/content-markup-reference.md
@@ -16,7 +16,7 @@
[Markdown](http://daringfireball.net/projects/markdown/) is a human-friendly syntax for formatting plain text. Our documentation is written with [GitHub Flavored Markdown](https://docs.github.com/en/github/writing-on-github/about-writing-and-formatting-on-github), a custom version of Markdown used across GitHub.
-This site's Markdown rendering is powered by the [`@github-docs/render-content`](https://github.com/docs/render-content) and [`hubdown`](https://github.com/electron/hubdown) npm packages, which are in turn built on the [`remark`](https://remark.js.org/) Markdown processor.
+This site's Markdown rendering is powered by the [`/lib/render-content`](/lib/render-content) and [`hubdown`](https://github.com/electron/hubdown) npm packages, which are in turn built on the [`remark`](https://remark.js.org/) Markdown processor.
## Callout tags
diff --git a/lib/render-content/README.md b/lib/render-content/README.md
new file mode 100644
index 000000000000..8be088bdc65d
--- /dev/null
+++ b/lib/render-content/README.md
@@ -0,0 +1,53 @@
+Markdown and Liquid rendering pipeline.
+
+## Usage
+
+```js
+const renderContent = require('.')
+
+const html = await renderContent(`
+# Beep
+{{ foo }}
+`, {
+ foo: 'bar'
+})
+```
+
+Creates:
+
+```html
+
+bar
+```
+
+## API
+
+### renderContent(markdown, context = {}, options = {})
+
+Render a string of `markdown` with optional `context`. Returns a `Promise`.
+
+Liquid will be looking for includes in `${process.cwd()}/includes`.
+
+Options:
+
+- `encodeEntities`: Encode html entities. Default: `false`.
+- `fileName`: File name for debugging purposes.
+- `textOnly`: Output text instead of html using [cheerio](https://ghub.io/cheerio).
+
+### .liquid
+
+The [Liquid](https://ghub.io/liquid) instance used internally.
+
+### Code block headers
+
+You can add a header to code blocks by adding the `{:copy}` annotation after the code fences:
+
+ ```js{:copy}
+ const copyMe = true
+ ```
+
+This renders:
+
+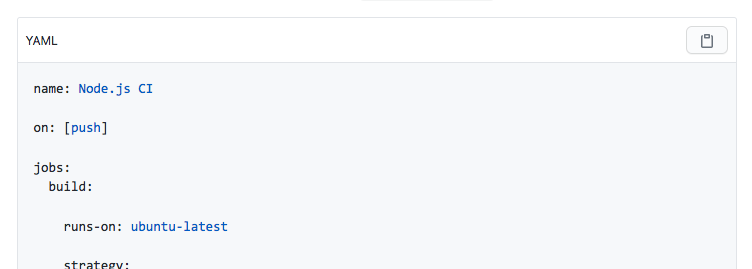
+
+The un-highlighted text is available as `button.js-btn-copy`'s `data-clipboard-text` attribute.
diff --git a/lib/render-content.js b/lib/render-content/index.js
similarity index 53%
rename from lib/render-content.js
rename to lib/render-content/index.js
index 99cc81072803..02068459b8ae 100644
--- a/lib/render-content.js
+++ b/lib/render-content/index.js
@@ -1,17 +1,17 @@
-const renderContent = require('@github-docs/render-content')
-const { ExtendedMarkdown, tags } = require('./liquid-tags/extended-markdown')
+const renderContent = require('./renderContent')
+const { ExtendedMarkdown, tags } = require('../liquid-tags/extended-markdown')
// Include custom tags like {% link_with_intro /article/foo %}
-renderContent.liquid.registerTag('liquid_tag', require('./liquid-tags/liquid-tag'))
-renderContent.liquid.registerTag('link', require('./liquid-tags/link'))
-renderContent.liquid.registerTag('link_with_intro', require('./liquid-tags/link-with-intro'))
-renderContent.liquid.registerTag('homepage_link_with_intro', require('./liquid-tags/homepage-link-with-intro'))
-renderContent.liquid.registerTag('link_in_list', require('./liquid-tags/link-in-list'))
-renderContent.liquid.registerTag('topic_link_in_list', require('./liquid-tags/topic-link-in-list'))
-renderContent.liquid.registerTag('link_with_short_title', require('./liquid-tags/link-with-short-title'))
-renderContent.liquid.registerTag('indented_data_reference', require('./liquid-tags/indented-data-reference'))
-renderContent.liquid.registerTag('data', require('./liquid-tags/data'))
-renderContent.liquid.registerTag('octicon', require('./liquid-tags/octicon'))
+renderContent.liquid.registerTag('liquid_tag', require('../liquid-tags/liquid-tag'))
+renderContent.liquid.registerTag('link', require('../liquid-tags/link'))
+renderContent.liquid.registerTag('link_with_intro', require('../liquid-tags/link-with-intro'))
+renderContent.liquid.registerTag('homepage_link_with_intro', require('../liquid-tags/homepage-link-with-intro'))
+renderContent.liquid.registerTag('link_in_list', require('../liquid-tags/link-in-list'))
+renderContent.liquid.registerTag('topic_link_in_list', require('../liquid-tags/topic-link-in-list'))
+renderContent.liquid.registerTag('link_with_short_title', require('../liquid-tags/link-with-short-title'))
+renderContent.liquid.registerTag('indented_data_reference', require('../liquid-tags/indented-data-reference'))
+renderContent.liquid.registerTag('data', require('../liquid-tags/data'))
+renderContent.liquid.registerTag('octicon', require('../liquid-tags/octicon'))
for (const tag in tags) {
// Register all the extended markdown tags, like {% note %} and {% warning %}
diff --git a/lib/render-content/liquid.js b/lib/render-content/liquid.js
new file mode 100644
index 000000000000..87106fe39764
--- /dev/null
+++ b/lib/render-content/liquid.js
@@ -0,0 +1,61 @@
+const Liquid = require('liquid')
+const semver = require('semver')
+const path = require('path')
+const engine = new Liquid.Engine()
+engine.registerFileSystem(
+ new Liquid.LocalFileSystem(path.join(process.cwd(), 'includes'))
+)
+
+// GHE versions are not valid SemVer, but can be coerced...
+// https://github.com/npm/node-semver#coercion
+
+Liquid.Condition.operators.ver_gt = (cond, left, right) => {
+ if (!matchesVersionString(left)) return false
+ if (!matchesVersionString(right)) return false
+
+ const [leftPlan, leftRelease] = splitVersion(left)
+ const [rightPlan, rightRelease] = splitVersion(right)
+
+ if (leftPlan !== rightPlan) return false
+
+ return semver.gt(semver.coerce(leftRelease), semver.coerce(rightRelease))
+}
+
+Liquid.Condition.operators.ver_lt = (cond, left, right) => {
+ if (!matchesVersionString(left)) return false
+ if (!matchesVersionString(right)) return false
+
+ const [leftPlan, leftRelease] = splitVersion(left)
+ const [rightPlan, rightRelease] = splitVersion(right)
+
+ if (leftPlan !== rightPlan) return false
+
+ return semver.lt(semver.coerce(leftRelease), semver.coerce(rightRelease))
+}
+
+module.exports = engine
+
+function matchesVersionString (input) {
+ return input && input.match(/^(?:[a-z](?:[a-z-]*[a-z])?@)?\d+(?:\.\d+)*/)
+}
+// Support new version formats where version = plan@release
+// e.g., enterprise-server@2.21, where enterprise-server is the plan and 2.21 is the release
+// e.g., free-pro-team@latest, where free-pro-team is the plan and latest is the release
+// in addition to legacy formats where the version passed is simply 2.21
+function splitVersion (version) {
+ // The default plan when working with versions is "enterprise-server".
+ // Default to that value here to support backward compatibility from before
+ // plans were explicitly included.
+ let plan = 'enterprise-server'
+ let release
+
+ const lastIndex = version.lastIndexOf('@')
+ if (lastIndex === -1) {
+ release = version
+ } else {
+ plan = version.slice(0, lastIndex)
+ release = version.slice(lastIndex + 1)
+ }
+
+ return [plan, release]
+}
diff --git a/lib/render-content/plugins/code-header.js b/lib/render-content/plugins/code-header.js
new file mode 100644
index 000000000000..bba0a806fc04
--- /dev/null
+++ b/lib/render-content/plugins/code-header.js
@@ -0,0 +1,138 @@
+const h = require('hastscript')
+const octicons = require('@primer/octicons')
+const parse5 = require('parse5')
+const fromParse5 = require('hast-util-from-parse5')
+
+const LANGUAGE_MAP = {
+ asp: 'ASP',
+ aspx: 'ASP',
+ 'aspx-vb': 'ASP',
+ as3: 'ActionScript',
+ apache: 'ApacheConf',
+ nasm: 'Assembly',
+ bat: 'Batchfile',
+ 'c#': 'C#',
+ csharp: 'C#',
+ c: 'C',
+ 'c++': 'C++',
+ cpp: 'C++',
+ chpl: 'Chapel',
+ coffee: 'CoffeeScript',
+ 'coffee-script': 'CoffeeScript',
+ cfm: 'ColdFusion',
+ 'common-lisp': 'Common Lisp',
+ lisp: 'Common Lisp',
+ dpatch: 'Darcs Patch',
+ dart: 'Dart',
+ elisp: 'Emacs Lisp',
+ emacs: 'Emacs Lisp',
+ 'emacs-lisp': 'Emacs Lisp',
+ pot: 'Gettext Catalog',
+ html: 'HTML',
+ xhtml: 'HTML',
+ 'html+erb': 'HTML+ERB',
+ erb: 'HTML+ERB',
+ irc: 'IRC log',
+ json: 'JSON',
+ jsp: 'Java Server Pages',
+ java: 'Java',
+ javascript: 'JavaScript',
+ js: 'JavaScript',
+ lhs: 'Literate Haskell',
+ 'literate-haskell': 'Literate Haskell',
+ objc: 'Objective-C',
+ openedge: 'OpenEdge ABL',
+ progress: 'OpenEdge ABL',
+ abl: 'OpenEdge ABL',
+ pir: 'Parrot Internal Representation',
+ posh: 'PowerShell',
+ puppet: 'Puppet',
+ 'pure-data': 'Pure Data',
+ raw: 'Raw token data',
+ rb: 'Ruby',
+ ruby: 'Ruby',
+ r: 'R',
+ scheme: 'Scheme',
+ bash: 'Shell',
+ sh: 'Shell',
+ shell: 'Shell',
+ zsh: 'Shell',
+ supercollider: 'SuperCollider',
+ tex: 'TeX',
+ ts: 'TypeScript',
+ vim: 'Vim script',
+ viml: 'Vim script',
+ rst: 'reStructuredText',
+ xbm: 'X BitMap',
+ xpm: 'X PixMap',
+ yaml: 'YAML',
+ yml: 'YAML',
+
+ // Unofficial languages
+ shellsession: 'Shell',
+ jsx: 'JSX'
+}
+
+const COPY_REGEX = /\{:copy\}$/
+
+/**
+ * Adds a bar above code blocks that shows the language and a copy button
+ */
+module.exports = function addCodeHeader (node) {
+ // Check if the language matches `lang{:copy}`
+ const hasCopy = node.lang && COPY_REGEX.test(node.lang)
+
+ if (hasCopy) {
+ // js{:copy} => js
+ node.lang = node.lang.replace(COPY_REGEX, '')
+ } else {
+ // It doesn't have the copy annotation, so don't add the header
+ return
+ }
+
+ // Display the language using the above map of `{ [shortCode]: language }`
+ const language = LANGUAGE_MAP[node.lang] || node.lang || 'Code'
+
+ const btnIconHtml = octicons.clippy.toSVG()
+ const btnIconAst = parse5.parse(String(btnIconHtml))
+ const btnIcon = fromParse5(btnIconAst, btnIconHtml)
+
+ // Need to create the header using Markdown AST utilities, to fit
+ // into the Unified processor ecosystem.
+ const header = h(
+ 'header',
+ {
+ class: [
+ 'd-flex',
+ 'flex-items-center',
+ 'flex-justify-between',
+ 'p-2',
+ 'text-small',
+ 'rounded-top-1',
+ 'border'
+ ]
+ },
+ [
+ h('span', language),
+ h(
+ 'button',
+ {
+ class: [
+ 'js-btn-copy',
+ 'btn',
+ 'btn-sm',
+ 'tooltipped',
+ 'tooltipped-nw'
+ ],
+ 'data-clipboard-text': node.value,
+ 'aria-label': 'Copy code to clipboard'
+ },
+ btnIcon
+ )
+ ]
+ )
+
+ return {
+ before: [header]
+ }
+}
diff --git a/lib/render-content/renderContent.js b/lib/render-content/renderContent.js
new file mode 100644
index 000000000000..a9a67ba84a58
--- /dev/null
+++ b/lib/render-content/renderContent.js
@@ -0,0 +1,106 @@
+const liquid = require('./liquid')
+const codeHeader = require('./plugins/code-header')
+const hubdown = require('hubdown')
+const remarkCodeExtra = require('remark-code-extra')
+const cheerio = require('cheerio')
+const Entities = require('html-entities').XmlEntities
+const entities = new Entities()
+const stripHtmlComments = require('strip-html-comments')
+
+// used below to remove extra newlines in TOC lists
+const endLine = '\r?\n'
+const blankLine = '\\s*?[\r\n]*'
+const startNextLine = '[^\\S\r\n]*?[-\\*] ?)\n?`, 'gm')
+
+// parse multiple times because some templates contain more templates. :]
+module.exports = async function renderContent (
+ template,
+ context = {},
+ options = {}
+) {
+ try {
+ // remove any newlines that precede html comments, then remove the comments
+ if (template) {
+ template = stripHtmlComments(template.replace(/\n Using cache
> ---> 0250ab3be9c5
> Successfully built 0250ab3be9c5
- ```
+ ```
4. Erstellen Sie einen Container:
@@ -54,7 +54,7 @@ Sie können ein Linux-Containerverwaltungstool zum Erstellen einer Pre-Receive-H
```shell
$ docker create --name pre-receive.alpine-3.3 pre-receive.alpine-3.3 /bin/true
- ```
+ ```
5. Exportieren Sie den Docker-Container in eine `gzip`-komprimierte `TAR`-Datei:
@@ -63,7 +63,7 @@ Sie können ein Linux-Containerverwaltungstool zum Erstellen einer Pre-Receive-H
```shell
$ docker export pre-receive.alpine-3.3 | gzip > alpine-3.3.tar.gz
- ```
+ ```
Diese Datei `alpine-3.3.tar.gz` kann auf die Appliance {% data variables.product.prodname_ghe_server %} hochgeladen werden.
@@ -76,9 +76,9 @@ Diese Datei `alpine-3.3.tar.gz` kann auf die Appliance {% data variables.product
2. Erstellen Sie eine `gzip`-komprimierte `TAR`-Datei des Verzeichnisses `chroot`.
- ```shell
- $ cd /path/to/chroot
- $ tar -czf /path/to/pre-receive-environment.tar.gz .
+ ```shell
+ $ cd /path/to/chroot
+ $ tar -czf /path/to/pre-receive-environment.tar.gz .
```
@@ -86,10 +86,11 @@ Diese Datei `alpine-3.3.tar.gz` kann auf die Appliance {% data variables.product
**Hinweise:**
- - Schließe keine führenden Verzeichnispfade von Dateien innerhalb des tar-Archivs ein, wie beispielsweise `/path/to/chroot`.
- - `/bin/sh` muss existieren und als Einstiegspunkt in die chroot-Umgebung ausführbar sein.
- - Im Gegensatz zu herkömmlichen Chroots ist das Verzeichnis `dev` für Vorempfang-Hooks nicht erforderlich.
-{% endnote %}
+ - Schließe keine führenden Verzeichnispfade von Dateien innerhalb des tar-Archivs ein, wie beispielsweise `/path/to/chroot`.
+ - `/bin/sh` muss existieren und als Einstiegspunkt in die chroot-Umgebung ausführbar sein.
+ - Im Gegensatz zu herkömmlichen Chroots ist das Verzeichnis `dev` für Vorempfang-Hooks nicht erforderlich.
+
+ {% endnote %}
Weitere Informationen zum Erstellen einer chroot-Umgebung finden Sie unter „[Chroot](https://wiki.debian.org/chroot)“ aus dem *Debian-Wiki*, „[BasicChroot](https://help.ubuntu.com/community/BasicChroot)“ aus dem *Hilfe-Wiki der Ubuntu-Community* oder „[Installing Alpine Linux in a chroot (Alpine Linux in einem chroot installieren](http://wiki.alpinelinux.org/wiki/Installing_Alpine_Linux_in_a_chroot)“ aus dem *Alpine Linux-Wiki*.
@@ -129,4 +130,4 @@ Weitere Informationen zum Erstellen einer chroot-Umgebung finden Sie unter „[C
```shell
admin@ghe-host:~$ ghe-hook-env-create AlpineTestEnv /home/admin/alpine-3.3.tar.gz
> Pre-receive hook environment 'AlpineTestEnv' (2) has been created.
- ```
+ ```
diff --git a/translations/de-DE/content/admin/policies/creating-a-pre-receive-hook-script.md b/translations/de-DE/content/admin/policies/creating-a-pre-receive-hook-script.md
index eb8e2b696dd9..e06b832e5f0c 100644
--- a/translations/de-DE/content/admin/policies/creating-a-pre-receive-hook-script.md
+++ b/translations/de-DE/content/admin/policies/creating-a-pre-receive-hook-script.md
@@ -70,19 +70,19 @@ Es wird empfohlen, Hooks in einem einzelnen Repository zu konsolidieren. Wenn da
```shell
$ sudo chmod +x SCRIPT_FILE.sh
- ```
- Stellen Sie für Windows-Benutzer sicher, dass die Skripts über Ausführungsberechtigungen verfügen:
+ ```
+ Stellen Sie für Windows-Benutzer sicher, dass die Skripts über Ausführungsberechtigungen verfügen:
- ```shell
- git update-index --chmod=+x SCRIPT_FILE.sh
- ```
+ ```shell
+ git update-index --chmod=+x SCRIPT_FILE.sh
+ ```
2. Committen und übertragen Sie Ihr vorgesehenes Pre-Receive-Hook-Repository auf der {% data variables.product.prodname_ghe_server %}-Instanz per Push-Vorgang.
```shell
$ git commit -m "YOUR COMMIT MESSAGE"
$ git push
- ```
+ ```
3. [Erstellen Sie den Pre-Receive-Hook](/enterprise/{{ currentVersion }}/admin/guides/developer-workflow/managing-pre-receive-hooks-on-the-github-enterprise-server-appliance/#creating-pre-receive-hooks) auf der {% data variables.product.prodname_ghe_server %}-Instanz.
@@ -93,40 +93,40 @@ Sie können ein Pre-Receive-Hook-Skript lokal testen, bevor Sie es auf Ihrer {%
2. Erstellen Sie eine Datei namens `Dockerfile.dev`, die Folgendes enthält:
- ```
- FROM gliderlabs/alpine:3.3
- RUN \
- apk add --no-cache git openssh bash && \
- ssh-keygen -A && \
- sed -i "s/#AuthorizedKeysFile/AuthorizedKeysFile/g" /etc/ssh/sshd_config && \
- adduser git -D -G root -h /home/git -s /bin/bash && \
- passwd -d git && \
- su git -c "mkdir /home/git/.ssh && \
- ssh-keygen -t ed25519 -f /home/git/.ssh/id_ed25519 -P '' && \
- mv /home/git/.ssh/id_ed25519.pub /home/git/.ssh/authorized_keys && \
- mkdir /home/git/test.git && \
- git --bare init /home/git/test.git"
-
- VOLUME ["/home/git/.ssh", "/home/git/test.git/hooks"]
- WORKDIR /home/git
-
- CMD ["/usr/sbin/sshd", "-D"]
- ```
+ ```
+ FROM gliderlabs/alpine:3.3
+ RUN \
+ apk add --no-cache git openssh bash && \
+ ssh-keygen -A && \
+ sed -i "s/#AuthorizedKeysFile/AuthorizedKeysFile/g" /etc/ssh/sshd_config && \
+ adduser git -D -G root -h /home/git -s /bin/bash && \
+ passwd -d git && \
+ su git -c "mkdir /home/git/.ssh && \
+ ssh-keygen -t ed25519 -f /home/git/.ssh/id_ed25519 -P '' && \
+ mv /home/git/.ssh/id_ed25519.pub /home/git/.ssh/authorized_keys && \
+ mkdir /home/git/test.git && \
+ git --bare init /home/git/test.git"
+
+ VOLUME ["/home/git/.ssh", "/home/git/test.git/hooks"]
+ WORKDIR /home/git
+
+ CMD ["/usr/sbin/sshd", "-D"]
+ ```
3. Erstellen Sie ein Pre-Receive-Testskript namens `always_reject.sh`. Dieses Beispielskript lehnt alle Push-Vorgänge ab, was zum Sperren eines Repositorys nützlich ist:
- ```
- #!/usr/bin/env bash
+ ```
+ #!/usr/bin/env bash
- echo "error: rejecting all pushes"
- exit 1
- ```
+ echo "error: rejecting all pushes"
+ exit 1
+ ```
4. Stellen Sie sicher, dass das Skript `always_reject.sh` über Ausführungsberechtigungen verfügt:
```shell
$ chmod +x always_reject.sh
- ```
+ ```
5. Erstellen Sie im Verzeichnis, in dem die `Dockerfile.dev` enthalten ist, ein Image:
@@ -149,32 +149,32 @@ Sie können ein Pre-Receive-Hook-Skript lokal testen, bevor Sie es auf Ihrer {%
....truncated output....
> Initialized empty Git repository in /home/git/test.git/
> Successfully built dd8610c24f82
- ```
+ ```
6. Führen Sie einen Datencontainer aus, der einen generierten SSH-Schlüssel enthält:
```shell
$ docker run --name data pre-receive.dev /bin/true
- ```
+ ```
7. Kopieren Sie den Pre-Receive-Hook `always_reject.sh` in den Datencontainer:
```shell
$ docker cp always_reject.sh data:/home/git/test.git/hooks/pre-receive
- ```
+ ```
8. Führen Sie einen Anwendungscontainer aus, der `sshd` und den Hook ausführt. Beachten Sie die zurückgegebene Container-ID.
```shell
$ docker run -d -p 52311:22 --volumes-from data pre-receive.dev
> 7f888bc700b8d23405dbcaf039e6c71d486793cad7d8ae4dd184f4a47000bc58
- ```
+ ```
9. Kopieren Sie den generierten SSH-Schlüssel aus dem Datencontainer auf den lokalen Computer:
```shell
$ docker cp data:/home/git/.ssh/id_ed25519 .
- ```
+ ```
10. Ändern Sie die Remote-Instanz eines Test-Repositorys, und übertragen Sie das Repository `test.git` per Push-Vorgang innerhalb des Docker-Containers. In diesem Beispiel wird `git@github.com:octocat/Hello-World.git` verwendet. Sie können jedoch auch andere Repositorys verwenden. In diesem Beispiel wird davon ausgegangen, dass Ihr lokaler Computer (127.0.0.1) den Port 52311 bindet. Sie können jedoch eine andere IP-Adresse verwenden, wenn Docker auf einem Remote-Computer ausgeführt wird.
@@ -193,9 +193,9 @@ Sie können ein Pre-Receive-Hook-Skript lokal testen, bevor Sie es auf Ihrer {%
> To git@192.168.99.100:test.git
> ! [remote rejected] main -> main (pre-receive hook declined)
> error: failed to push some refs to 'git@192.168.99.100:test.git'
- ```
+ ```
- Beachten Sie, dass der Push abgelehnt wurde, nachdem Sie den Pre-Receive-Hook ausgeführt und die Ausgabe des Skripts wiedergegeben haben.
+ Beachten Sie, dass der Push abgelehnt wurde, nachdem Sie den Pre-Receive-Hook ausgeführt und die Ausgabe des Skripts wiedergegeben haben.
### Weiterführende Informationen
- „[Git anpassen – eine Git-erzwungene Beispielrichtlinie](https://git-scm.com/book/en/v2/Customizing-Git-An-Example-Git-Enforced-Policy)“ von der *Pro Git-Website*
diff --git a/translations/de-DE/content/admin/policies/enforcing-repository-management-policies-in-your-enterprise.md b/translations/de-DE/content/admin/policies/enforcing-repository-management-policies-in-your-enterprise.md
index dd86ed62b974..a6fd918166ae 100644
--- a/translations/de-DE/content/admin/policies/enforcing-repository-management-policies-in-your-enterprise.md
+++ b/translations/de-DE/content/admin/policies/enforcing-repository-management-policies-in-your-enterprise.md
@@ -34,7 +34,7 @@ versions:
Each time someone creates a new repository on your enterprise, that person must choose a visibility for the repository. When you configure a default visibility setting for the enterprise, you choose which visibility is selected by default. Weitere Informationen zu Repository-Sichtbarkeiten findest Du unter „[Informationen zur Sichtbarkeit eines Repositorys](/github/creating-cloning-and-archiving-repositories/about-repository-visibility)“.
-Wenn ein Site-Administrator Mitgliedern das Erstellen bestimmter Arten Repositorys verwehrt, werden Mitglieder nicht in der Lage sein, ein Repository dieser Art zu erstellen, selbst wenn die Einstellung zur Sichtbarkeit diesen Typ als Standard vorgibt. For more information, see "[Setting a policy for repository creation](#setting-a-policy-for-repository-creation)."
+If an enterprise owner disallows members from creating certain types of repositories, members will not be able to create that type of repository even if the visibility setting defaults to that type. For more information, see "[Setting a policy for repository creation](#setting-a-policy-for-repository-creation)."
{% data reusables.enterprise-accounts.access-enterprise %}
{% if currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
@@ -49,9 +49,9 @@ Wenn ein Site-Administrator Mitgliedern das Erstellen bestimmter Arten Repositor
### Setting a policy for changing a repository's visibility
-Wenn Sie Mitglieder daran hindern, die Sichtbarkeit des Repositorys zu ändern, können nur Websiteadministratoren öffentliche Repositorys als privat oder private Repositorys als öffentlich festlegen.
+When you prevent members from changing repository visibility, only enterprise owners can change the visibility of a repository.
-Falls ein Websiteadministrator die Möglichkeit der Repository-Erstellung auf Organisationsinhaber beschränkt hat, können Mitglieder die Sichtbarkeit eines Repositorys nicht ändern. Hat ein Websiteadministrator dagegen die Möglichkeit von Mitgliedern auf die Erstellung privater Repositorys beschränkt, können Mitglieder die Sichtbarkeit eines Repositorys von öffentlich auf privat festlegen. For more information, see "[Setting a policy for repository creation](#setting-a-policy-for-repository-creation)."
+If an enterprise owner has restricted repository creation to organization owners only, then members will not be able to change repository visibility. If an enterprise owner has restricted member repository creation to private repositories only, then members will only be able to change the visibility of a repository to private. For more information, see "[Setting a policy for repository creation](#setting-a-policy-for-repository-creation)."
{% data reusables.enterprise-accounts.access-enterprise %}
{% data reusables.enterprise-accounts.policies-tab %}
@@ -75,6 +75,15 @@ Falls ein Websiteadministrator die Möglichkeit der Repository-Erstellung auf Or
6. Wählen Sie im Dropdownmenü unter „Repository creation“ (Repository-Erstellung) eine Richtlinie aus. 
{% endif %}
+### Eine Richtlinie zum Forken privater oder interner Repositorys erzwingen
+
+Across all organizations owned by your enterprise, you can allow people with access to a private or internal repository to fork the repository, never allow forking of private or internal repositories, or allow owners to administer the setting on the organization level.
+
+{% data reusables.enterprise-accounts.access-enterprise %}
+{% data reusables.enterprise-accounts.policies-tab %}
+3. Lies auf der Registerkarte **Repository policies** (Repository-Richtlinien) unter „Repository forking“ (Repository-Forking) die Informationen zum Ändern der Einstellung. {% data reusables.enterprise-accounts.view-current-policy-config-orgs %}
+4. Wähle im Dropdownmenü unter „Repository forking“ (Repository-Forking) eine Richtlinie aus. 
+
### Setting a policy for repository deletion and transfer
{% data reusables.enterprise-accounts.access-enterprise %}
@@ -166,6 +175,8 @@ Sie können die standardmäßig übernommenen Einstellungen überschreiben, inde
- **Block to the default branch** (Übertragung an den Standardbranch blockieren), damit die an den Standardbranch übertragenen erzwungenen Push-Vorgänge blockiert werden. 
6. Wählen Sie optional **Enforce on all repositories** (Auf allen Repositorys erzwingen) aus, um Repository-spezifische Einstellungen zu überschreiben. Note that this will **not** override an enterprise-wide policy. 
+{% if enterpriseServerVersions contains currentVersion %}
+
### Configuring anonymous Git read access
{% data reusables.enterprise_user_management.disclaimer-for-git-read-access %}
@@ -192,7 +203,6 @@ If necessary, you can prevent repository administrators from changing anonymous
4. Klicken Sie unter „Anonymous Git read access“ (Anonymer Git-Lesezugriff) auf das Dropdownmenü, und klicken Sie auf **Enabled** (Aktiviert). 
3. Optionally, to prevent repository admins from changing anonymous Git read access settings in all repositories on your enterprise, select **Prevent repository admins from changing anonymous Git read access**. 
-{% if enterpriseServerVersions contains currentVersion %}
#### Setting anonymous Git read access for a specific repository
{% data reusables.enterprise_site_admin_settings.access-settings %}
@@ -203,6 +213,7 @@ If necessary, you can prevent repository administrators from changing anonymous
6. Klicken Sie unter „Danger Zone“ (Gefahrenzone) neben „Enable Anonymous Git read access“ (Anonymen Git-Lesezugriff aktivieren) auf **Enable** (Aktivieren). 
7. Überprüfen Sie die Änderungen. Klicken Sie zur Bestätigung auf **Yes, enable anonymous Git read access** (Ja, anonymen Git-Lesezugriff aktivieren). 
8. Aktivieren Sie optional **Prevent repository admins from changing anonymous Git read access** (Repository-Administratoren daran hindern, den anonymen Git-Lesezugriff zu ändern), um Repository-Administratoren daran zu hindern, diese Einstellung für dieses Repository zu ändern. 
+
{% endif %}
{% if currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
diff --git a/translations/de-DE/content/admin/release-notes.md b/translations/de-DE/content/admin/release-notes.md
new file mode 100644
index 000000000000..d312be458ea8
--- /dev/null
+++ b/translations/de-DE/content/admin/release-notes.md
@@ -0,0 +1,8 @@
+---
+title: Release notes
+intro: The release notes for {{ allVersions[currentVersion].versionTitle }}.
+layout: release-notes
+versions:
+ enterprise-server: '*'
+---
+
diff --git a/translations/de-DE/content/admin/user-management/audited-actions.md b/translations/de-DE/content/admin/user-management/audited-actions.md
index cfde2509ad69..245261643bea 100644
--- a/translations/de-DE/content/admin/user-management/audited-actions.md
+++ b/translations/de-DE/content/admin/user-management/audited-actions.md
@@ -36,12 +36,12 @@ versions:
#### Enterprise configuration settings
-| Name | Beschreibung |
-| -------------------------------------------------------:| ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `business.update_member_repository_creation_permission` | A site admin restricts repository creation in organizations in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#setting-a-policy-for-repository-creation)." |
-| `business.clear_members_can_create_repos` | A site admin clears a restriction on repository creation in organizations in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#setting-a-policy-for-repository-creation)." |
-| `enterprise.config.lock_anonymous_git_access` | A site admin locks anonymous Git read access to prevent repository admins from changing existing anonymous Git read access settings for repositories in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#configuring-anonymous-git-read-access)." |
-| `enterprise.config.unlock_anonymous_git_access` | A site admin unlocks anonymous Git read access to allow repository admins to change existing anonymous Git read access settings for repositories in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#configuring-anonymous-git-read-access)." |
+| Name | Beschreibung |
+| -------------------------------------------------------:| -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `business.update_member_repository_creation_permission` | A site admin restricts repository creation in organizations in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#setting-a-policy-for-repository-creation)." |
+| `business.clear_members_can_create_repos` | A site admin clears a restriction on repository creation in organizations in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#setting-a-policy-for-repository-creation)."{% if enterpriseServerVersions contains currentVersion %}
+| `enterprise.config.lock_anonymous_git_access` | A site admin locks anonymous Git read access to prevent repository admins from changing existing anonymous Git read access settings for repositories in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#configuring-anonymous-git-read-access)." |
+| `enterprise.config.unlock_anonymous_git_access` | A site admin unlocks anonymous Git read access to allow repository admins to change existing anonymous Git read access settings for repositories in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#configuring-anonymous-git-read-access)."{% endif %}
#### Issues und Pull Requests
@@ -79,7 +79,7 @@ versions:
| Name | Beschreibung |
| ------------------------------------------:| -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `repo.access` | Ein privates Repository wurde als öffentlich festgelegt, oder ein öffentliches Repository wurde als privat festgelegt. |
+| `repo.access` | The visibility of a repository changed to private{% if enterpriseServerVersions contains currentVersion %}, public,{% endif %} or internal. |
| `repo.archive` | Ein Repository wurde archiviert. For more information, see "[Archiving a {% data variables.product.prodname_dotcom %} repository](/github/creating-cloning-and-archiving-repositories/archiving-a-github-repository)." |
| `repo.add_member` | Einem Repository wurde ein Mitarbeiter hinzugefügt. |
| `repo.config` | Ein Websiteadministrator hat erzwungene Push-Vorgänge blockiert. Weitere Informationen finden Sie unter „[Erzwungene Push-Vorgänge an ein Repository blockieren](/enterprise/{{ currentVersion }}/admin/guides/developer-workflow/blocking-force-pushes-to-a-repository/)“. |
@@ -89,11 +89,11 @@ versions:
| `repo.rename` | Ein Repository wurde umbenannt. |
| `repo.transfer` | Ein Benutzer hat eine Anfrage akzeptiert, ein übertragenes Repository zu empfangen. |
| `repo.transfer_start` | Ein Benutzer hat eine Anfrage gesendet, ein Repository an einen anderen Benutzer oder an eine andere Organisation zu übertragen. |
-| `repo.unarchive` | Die Archivierung eines Repositorys wurde aufgehoben. For more information, see "[Archiving a {% data variables.product.prodname_dotcom %} repository](/github/creating-cloning-and-archiving-repositories/archiving-a-github-repository)." |
-| `repo.config.disable_anonymous_git_access` | Der anonyme Git-Lesezugriff ist für ein öffentliches Repository deaktiviert. Weitere Informationen finden Sie unter „[Anonymen Git-Lesezugriff für ein Repository aktivieren](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)“. |
-| `repo.config.enable_anonymous_git_access` | Der anonyme Git-Lesezugriff ist für ein öffentliches Repository aktiviert. Weitere Informationen finden Sie unter „[Anonymen Git-Lesezugriff für ein Repository aktivieren](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)“. |
+| `repo.unarchive` | Die Archivierung eines Repositorys wurde aufgehoben. For more information, see "[Archiving a {% data variables.product.prodname_dotcom %} repository](/github/creating-cloning-and-archiving-repositories/archiving-a-github-repository)."{% if enterpriseServerVersions contains currentVersion %}
+| `repo.config.disable_anonymous_git_access` | Anonymous Git read access is disabled for a repository. Weitere Informationen finden Sie unter „[Anonymen Git-Lesezugriff für ein Repository aktivieren](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)“. |
+| `repo.config.enable_anonymous_git_access` | Anonymous Git read access is enabled for a repository. Weitere Informationen finden Sie unter „[Anonymen Git-Lesezugriff für ein Repository aktivieren](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)“. |
| `repo.config.lock_anonymous_git_access` | Die Einstellung für den anonymen Git-Lesezugriff eines Repositorys ist gesperrt, wodurch Repository-Administratoren daran gehindert werden, diese Einstellung zu ändern (zu aktivieren oder zu deaktivieren). Weitere Informationen finden Sie unter „[Änderung des anonymen Git-Lesezugriffs durch Benutzer verhindern](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)“. |
-| `repo.config.unlock_anonymous_git_access` | Die Einstellung für den anonymen Git-Lesezugriff ist entsperrt, wodurch Repository-Administratoren diese Einstellung ändern (aktivieren oder deaktivieren) können. Weitere Informationen finden Sie unter „[Änderung des anonymen Git-Lesezugriffs durch Benutzer verhindern](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)“. |
+| `repo.config.unlock_anonymous_git_access` | Die Einstellung für den anonymen Git-Lesezugriff ist entsperrt, wodurch Repository-Administratoren diese Einstellung ändern (aktivieren oder deaktivieren) können. For more information, see "[Preventing users from changing anonymous Git read access](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)."{% endif %}
#### Websiteadministratortools
diff --git a/translations/de-DE/content/admin/user-management/managing-organizations-in-your-enterprise.md b/translations/de-DE/content/admin/user-management/managing-organizations-in-your-enterprise.md
index b304f19c4dd1..916a60afb3a1 100644
--- a/translations/de-DE/content/admin/user-management/managing-organizations-in-your-enterprise.md
+++ b/translations/de-DE/content/admin/user-management/managing-organizations-in-your-enterprise.md
@@ -5,7 +5,7 @@ redirect_from:
- /enterprise/admin/categories/admin-bootcamp/
- /enterprise/admin/user-management/organizations-and-teams
- /enterprise/admin/user-management/managing-organizations-in-your-enterprise
-intro: 'Organisationen eignen sich ideal zum Erstellen von getrennten Benutzergruppen in Ihrem Unternehmen, beispielsweise Abteilungen oder Gruppen, die an ähnlichen Projekten arbeiten. Benutzer in anderen Organisationen können auf öffentliche Repositorys zugreifen, die zu einer Organisation gehören. Demgegenüber können auf private Repositorys nur Mitglieder der Organisation zugreifen.'
+intro: 'Organisationen eignen sich ideal zum Erstellen von getrennten Benutzergruppen in Ihrem Unternehmen, beispielsweise Abteilungen oder Gruppen, die an ähnlichen Projekten arbeiten. {% if currentVersion == "github-ae@latest" %}Internal{% else %}Public and internal{% endif %} repositories that belong to an organization are accessible to users in other organizations, while private repositories are inaccessible to anyone but members of the organization that are granted access.'
mapTopic: true
versions:
enterprise-server: '*'
diff --git a/translations/de-DE/content/developers/apps/authorizing-oauth-apps.md b/translations/de-DE/content/developers/apps/authorizing-oauth-apps.md
index dd0a620a7d56..375d0be5852a 100644
--- a/translations/de-DE/content/developers/apps/authorizing-oauth-apps.md
+++ b/translations/de-DE/content/developers/apps/authorizing-oauth-apps.md
@@ -114,11 +114,13 @@ curl -H "Authorization: token OAUTH-TOKEN" {% data variables.product.api_url_pre
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
### Device flow
+{% if currentVersion ver_lt "enterprise-server@3.1" %}
{% note %}
-**Note:** The device flow is in public beta and subject to change.{% if currentVersion == "free-pro-team@latest" %} To enable this beta feature, see "[Activating beta features for apps](/developers/apps/activating-beta-features-for-apps)."{% endif %}
+**Note:** The device flow is in public beta and subject to change.
{% endnote %}
+{% endif %}
The device flow allows you to authorize users for a headless app, such as a CLI tool or Git credential manager.
diff --git a/translations/de-DE/content/developers/apps/identifying-and-authorizing-users-for-github-apps.md b/translations/de-DE/content/developers/apps/identifying-and-authorizing-users-for-github-apps.md
index 0ecffcb6b4de..734c3965dee9 100644
--- a/translations/de-DE/content/developers/apps/identifying-and-authorizing-users-for-github-apps.md
+++ b/translations/de-DE/content/developers/apps/identifying-and-authorizing-users-for-github-apps.md
@@ -123,11 +123,13 @@ curl -H "Authorization: token OAUTH-TOKEN" {% data variables.product.api_url_pre
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
### Device flow
+{% if currentVersion ver_lt "enterprise-server@3.1" %}
{% note %}
-**Note:** The device flow is in public beta and subject to change.{% if currentVersion == "free-pro-team@latest" %} To enable this beta feature, see "[Activating beta features for apps](/developers/apps/activating-beta-features-for-apps)."{% endif %}
+**Note:** The device flow is in public beta and subject to change.
{% endnote %}
+{% endif %}
The device flow allows you to authorize users for a headless app, such as a CLI tool or Git credential manager.
diff --git a/translations/de-DE/content/developers/webhooks-and-events/webhook-events-and-payloads.md b/translations/de-DE/content/developers/webhooks-and-events/webhook-events-and-payloads.md
index 7e26e5709a54..a69238f4bd08 100644
--- a/translations/de-DE/content/developers/webhooks-and-events/webhook-events-and-payloads.md
+++ b/translations/de-DE/content/developers/webhooks-and-events/webhook-events-and-payloads.md
@@ -1288,7 +1288,7 @@ The event’s actor is the [user](/v3/users/) who starred a repository, and the
{{ webhookPayloadsForCurrentVersion.watch.started }}
-{% if currentVersion == "free-pro-team@latest" %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
### workflow_dispatch
This event occurs when someone triggers a workflow run on GitHub or sends a `POST` request to the "[Create a workflow dispatch event](/rest/reference/actions/#create-a-workflow-dispatch-event)" endpoint. Weitere Informationen findest Du unter "[Ereignisse, die Workflows auslösen](/actions/reference/events-that-trigger-workflows#workflow_dispatch)."
@@ -1302,9 +1302,10 @@ This event occurs when someone triggers a workflow run on GitHub or sends a `POS
{{ webhookPayloadsForCurrentVersion.workflow_dispatch }}
{% endif %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %}
### workflow_run
-When a {% data variables.product.prodname_actions %} workflow run is requested or completed. Weitere Informationen findest Du unter "[Ereignisse, die Workflows auslösen](/actions/reference/events-that-trigger-workflows#workflow_run)."
+When a {% data variables.product.prodname_actions %} workflow run is requested or completed. For more information, see "[Events that trigger workflows](/actions/reference/events-that-trigger-workflows#workflow_run)."
#### Availability
@@ -1322,3 +1323,4 @@ When a {% data variables.product.prodname_actions %} workflow run is requested o
#### Webhook payload example
{{ webhookPayloadsForCurrentVersion.workflow_run }}
+{% endif %}
diff --git a/translations/de-DE/content/github/administering-a-repository/about-secret-scanning.md b/translations/de-DE/content/github/administering-a-repository/about-secret-scanning.md
index d151bd6a2101..188b6b5f8d33 100644
--- a/translations/de-DE/content/github/administering-a-repository/about-secret-scanning.md
+++ b/translations/de-DE/content/github/administering-a-repository/about-secret-scanning.md
@@ -18,6 +18,8 @@ Dienstleister können mit {% data variables.product.company_short %} zusammenarb
### Informationen über {% data variables.product.prodname_secret_scanning %} für öffentliche Repositorys
+ {% data variables.product.prodname_secret_scanning_caps %} is automatically enabled on public repositories, where it scans code for secrets, to check for known secret formats. When a match of your secret format is found in a public repository, {% data variables.product.company_short %} doesn't publicly disclose the information as an alert, but instead sends a payload to an HTTP endpoint of your choice. For an overview of how secret scanning works on public repositories, see "[Secret scanning](/developers/overview/secret-scanning)."
+
Wenn Du in ein öffentliches Repository überträgst, wird {% data variables.product.product_name %} den Inhalt des Commit auf Geheimnisse durchsuchen. Wenn Du ein privates Repository auf öffentlich umstellst, wird {% data variables.product.product_name %} das gesamte Repository nach Geheimnissen durchsuchen.
Wenn {% data variables.product.prodname_secret_scanning %} einen Satz von Anmeldeinformationen erkennt, benachrichtigen wir den Dienstanbieter, der das Geheimnis ausgegeben hat. Der Dienstanbieter prüft die Anmeldeinformationen und entscheidet dann, ob er das Geheimnis widerrufen, ein neues Geheimnis ausstellen oder sich direkt an Dich wenden soll, was von den damit verbundenen Risiken für Dich oder den Dienstleister abhängt.
@@ -65,6 +67,8 @@ Wenn {% data variables.product.prodname_secret_scanning %} einen Satz von Anmeld
{% data reusables.secret-scanning.beta %}
+If you're a repository administrator or an organization owner, you can enable {% data variables.product.prodname_secret_scanning %} for private repositories that are owned by organizations. You can enable {% data variables.product.prodname_secret_scanning %} for all your repositories, or for all new repositories within your organization. {% data variables.product.prodname_secret_scanning_caps %} is not available for user account-owned private repositories. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository)" and "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)."
+
Wenn Du Commits in ein privates Repository überträgst, das {% data variables.product.prodname_secret_scanning %} aktiviert hat, wird {% data variables.product.product_name %} den Inhalt des Commits nach Geheimnissen durchsuchen.
Wenn {% data variables.product.prodname_secret_scanning %} ein Geheimnis in einem privaten Repository entdeckt, wird {% data variables.product.prodname_dotcom %} Warnungen senden.
@@ -73,6 +77,8 @@ Wenn {% data variables.product.prodname_secret_scanning %} ein Geheimnis in eine
- {% data variables.product.prodname_dotcom %} zeigt eine Warnung im Repository an. Weitere Informationen findest Du unter „[Warnungen von {% data variables.product.prodname_secret_scanning %} verwalten](/github/administering-a-repository/managing-alerts-from-secret-scanning)."
+Repository administrators and organization owners can grant users and team access to {% data variables.product.prodname_secret_scanning %} alerts. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-security-alerts)."
+
{% data variables.product.product_name %} durchsucht derzeit private Repositorys nach Geheimnissen, die von den folgenden Dienstanbietern veröffentlicht wurden.
- Adafruit
diff --git a/translations/de-DE/content/github/administering-a-repository/classifying-your-repository-with-topics.md b/translations/de-DE/content/github/administering-a-repository/classifying-your-repository-with-topics.md
index 3f48a46123e2..5cd352637aa1 100644
--- a/translations/de-DE/content/github/administering-a-repository/classifying-your-repository-with-topics.md
+++ b/translations/de-DE/content/github/administering-a-repository/classifying-your-repository-with-topics.md
@@ -22,7 +22,7 @@ Rufe https://github.com/topics/ auf, um die am häufigsten verwendeten Themen zu
Repository-Administratoren können beliebige Themen zu einem Repository hinzufügen. Helpful topics to classify a repository include the repository's intended purpose, subject area, community, or language.{% if currentVersion == "free-pro-team@latest" %} Additionally, {% data variables.product.product_name %} analyzes public repository content and generates suggested topics that repository admins can accept or reject. Die Inhalte privater Repositorys werden nicht analysiert, und es gibt keine Themenvorschläge für private Repositorys.{% endif %}
-Öffentliche und private Repositorys können Themen haben, aber in den Resultaten der Themensuche wirst Du nur die privaten Repositorys sehen, auf die Du Zugriff hast.
+{% if currentVersion == "github-ae@latest" %}Internal {% else %}Public, internal, {% endif %}and private repositories can have topics, although you will only see private repositories that you have access to in topic search results.
Du kannst nach Repositorys suchen, die mit einem bestimmten Thema verknüpft sind. Weitere Informationen finden Sie unter „[Nach Repositorys suchen](/articles/searching-for-repositories/#search-by-topic)“. Sie können auch nach einer Liste von Themen auf {% data variables.product.product_name %} suchen. Weitere Informationen findest Du unter „[Themen suchen](/articles/searching-topics).“
diff --git a/translations/de-DE/content/github/administering-a-repository/customizing-your-repositorys-social-media-preview.md b/translations/de-DE/content/github/administering-a-repository/customizing-your-repositorys-social-media-preview.md
index 06a99ba77352..82b0c53da18b 100644
--- a/translations/de-DE/content/github/administering-a-repository/customizing-your-repositorys-social-media-preview.md
+++ b/translations/de-DE/content/github/administering-a-repository/customizing-your-repositorys-social-media-preview.md
@@ -11,7 +11,7 @@ versions:
Wenn Du noch kein Bild hinzugefügt hast, werden sich Repository-Links erweitern, um grundlegende Informationen zum Repository sowie den Avatar des Inhabers anzeigen. Durch das Hinzufügen eines Bildes zu Deinem Repository vereinfachst Du die Identifizierung Deines Projekts auf den verschiedenen Social-Media-Kanälen.
-Du kannst ein Bild in ein privates Repository hochladen, aber Dein Bild kann nur von einem öffentlichen Repository her geteilt werden.
+{% if currentVersion != "github-ae@latest" %}You can upload an image to a private repository, but your image can only be shared from a public repository.{% endif %}
{% tip %}
Tipp: Dein Bild sollte eine PNG-, JPG- oder GIF-Datei mit weniger als 1 MB sein. Für eine optimale Darstellung empfehlen wir eine Bildgröße von 640 x 320 Pixeln.
diff --git a/translations/de-DE/content/github/administering-a-repository/deleting-a-repository.md b/translations/de-DE/content/github/administering-a-repository/deleting-a-repository.md
index bc222168f680..1ba37f21dc10 100644
--- a/translations/de-DE/content/github/administering-a-repository/deleting-a-repository.md
+++ b/translations/de-DE/content/github/administering-a-repository/deleting-a-repository.md
@@ -13,17 +13,16 @@ versions:
{% data reusables.organizations.owners-and-admins-can %} ein Organisations-Repository löschen. Wenn **Allow members to delete or transfer repositories for this organization** (Mitgliedern das Löschen oder Übertragen von Repositorys für diese Organisation erlauben) deaktiviert wurde, können nur Organisationsinhaber Repositorys der Organisation löschen. {% data reusables.organizations.new-repo-permissions-more-info %}
-{% if currentVersion == "free-pro-team@latest" %}
+{% if currentVersion != "github-ae@latest" %}Deleting a public repository will not delete any forks of the repository.{% endif %}
+
{% warning %}
-**Warnung**: Wenn Du ein Repository löschst, werden die Releaseanhänge und Teamberechtigungen **dauerhaft** gelöscht. Diese Aktion **kann nicht** rückgängig gemacht werden.
+**Warnings**:
-{% endwarning %}
-{% endif %}
+- Deleting a repository will **permanently** delete release attachments and team permissions. Diese Aktion **kann nicht** rückgängig gemacht werden.
+- Deleting a private {% if currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" %}or internal {% endif %}repository will delete all forks of the repository.
-Beachte auch Folgendes:
-- Beim Löschen eines privaten Repositorys werden all seine Forks ebenfalls gelöscht.
-- Beim Löschen eines öffentlichen Repositorys werden seine Forks nicht gelöscht.
+{% endwarning %}
{% if currentVersion == "free-pro-team@latest" %}
Bestimmte gelöschte Repositorys können innerhalb von 90 Tagen wiederhergestellt werden. Weitere Informationen findest Du unter „[Ein gelöschtes Repository wiederherstellen](/articles/restoring-a-deleted-repository).“
diff --git a/translations/de-DE/content/github/administering-a-repository/enabling-anonymous-git-read-access-for-a-repository.md b/translations/de-DE/content/github/administering-a-repository/enabling-anonymous-git-read-access-for-a-repository.md
index 102a7f8936fc..5ce36eebfc81 100644
--- a/translations/de-DE/content/github/administering-a-repository/enabling-anonymous-git-read-access-for-a-repository.md
+++ b/translations/de-DE/content/github/administering-a-repository/enabling-anonymous-git-read-access-for-a-repository.md
@@ -5,7 +5,6 @@ redirect_from:
- /articles/enabling-anonymous-git-read-access-for-a-repository
versions:
enterprise-server: '*'
- github-ae: '*'
---
Repository-Administratoren können die Einstellung für den anonymen Git-Lesezugriff für ein bestimmtes Repository ändern, wenn folgende Voraussetzungen erfüllt sind:
diff --git a/translations/de-DE/content/github/administering-a-repository/managing-repository-settings.md b/translations/de-DE/content/github/administering-a-repository/managing-repository-settings.md
index f6f6b61444ad..12b130163430 100644
--- a/translations/de-DE/content/github/administering-a-repository/managing-repository-settings.md
+++ b/translations/de-DE/content/github/administering-a-repository/managing-repository-settings.md
@@ -1,6 +1,6 @@
---
title: Repository-Einstellungen verwalten
-intro: 'Repository-Administratoren und Organisationsinhaber können mehrere Einstellungen ändern, beispielsweise die Namen und die Inhaberschaft eines Repositorys und die Sichtbarkeit (privat oder öffentlich) eines Repositorys. Sie können ein Repository auch löschen.'
+intro: 'Repository administrators and organization owners can change settings for a repository, like the name, ownership, and visibility, or delete the repository.'
mapTopic: true
redirect_from:
- /articles/managing-repository-settings
diff --git a/translations/de-DE/content/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository.md b/translations/de-DE/content/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository.md
index d4d6862136f4..8440bfee12a8 100644
--- a/translations/de-DE/content/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository.md
+++ b/translations/de-DE/content/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository.md
@@ -22,28 +22,28 @@ versions:
{% data reusables.repositories.navigate-to-security-and-analysis %}
4. Under "Configure security and analysis features", to the right of the feature, click **Disable** or **Enable**. 
-### Granting access to {% data variables.product.prodname_dependabot_alerts %}
+### Zugriff auf Sicherheitsmeldungen gewähren
-After you enable {% data variables.product.prodname_dependabot_alerts %} for a repository in an organization, organization owners and repository administrators can view the alerts by default. You can give additional teams and people access to the alerts for a repository.
+After you enable {% data variables.product.prodname_dependabot %} or {% data variables.product.prodname_secret_scanning %} alerts for a repository in an organization, organization owners and repository administrators can view the alerts by default. You can give additional teams and people access to the alerts for a repository.
{% note %}
-Organization owners and repository administrators can only grant access to view {% data variables.product.prodname_dependabot_alerts %} to people or teams who have write access to the repo.
+Organization owners and repository administrators can only grant access to view security alerts, such as {% data variables.product.prodname_dependabot %} and {% data variables.product.prodname_secret_scanning %} alerts, to people or teams who have write access to the repo.
{% endnote %}
{% data reusables.repositories.navigate-to-repo %}
{% data reusables.repositories.sidebar-settings %}
{% data reusables.repositories.navigate-to-security-and-analysis %}
-4. Under "Dependabot alerts", in the search field, start typing the name of the person or team you'd like to find, then click a name in the list of matches. 
-5. Klicke auf **Save changes** (Änderungen speichern). 
+4. Under "Access to alerts", in the search field, start typing the name of the person or team you'd like to find, then click a name in the list of matches. 
+5. Klicke auf **Save changes** (Änderungen speichern). 
-### Removing access to {% data variables.product.prodname_dependabot_alerts %}
+### Zugriff auf Sicherheitsmeldungen entfernen
{% data reusables.repositories.navigate-to-repo %}
{% data reusables.repositories.sidebar-settings %}
{% data reusables.repositories.navigate-to-security-and-analysis %}
-4. Under "Dependabot alerts", to the right of the person or team whose access you'd like to remove, click {% octicon "x" aria-label="X symbol" %}. 
+4. Under "Access to alerts", to the right of the person or team whose access you'd like to remove, click {% octicon "x" aria-label="X symbol" %}. 
### Weiterführende Informationen
diff --git a/translations/de-DE/content/github/administering-a-repository/setting-repository-visibility.md b/translations/de-DE/content/github/administering-a-repository/setting-repository-visibility.md
index 6d2402623367..5f1b998c9c49 100644
--- a/translations/de-DE/content/github/administering-a-repository/setting-repository-visibility.md
+++ b/translations/de-DE/content/github/administering-a-repository/setting-repository-visibility.md
@@ -19,17 +19,36 @@ Organisationsinhaber können die Möglichkeit, die Sichtbarkeit des Repositorys
We recommend reviewing the following caveats before you change the visibility of a repository.
#### Repository als privat festlegen
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+* {% data variables.product.product_name %} will detach public forks of the public repository and put them into a new network. Public forks are not made private.{% endif %}
+* If you change a repository's visibility from internal to private, {% data variables.product.prodname_dotcom %} will remove forks that belong to any user without access to the newly private repository. {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}The visibility of any forks will also change to private.{% elsif currentVersion == "github-ae@latest" %}If the internal repository has any forks, the visibility of the forks is already private.{% endif %} For more information, see "[What happens to forks when a repository is deleted or changes visibility?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility)"{% if currentVersion == "free-pro-team@latest" %}
+* If you're using {% data variables.product.prodname_free_user %} for user accounts or organizations, some features won't be available in the repository after you change the visibility to private. {% data reusables.gated-features.more-info %}{% endif %}
+* Any published {% data variables.product.prodname_pages %} site will be automatically unpublished.{% if currentVersion == "free-pro-team@latest" %} If you added a custom domain to the {% data variables.product.prodname_pages %} site, you should remove or update your DNS records before making the repository private, to avoid the risk of a domain takeover. For more information, see "[Managing a custom domain for your {% data variables.product.prodname_pages %} site](/articles/managing-a-custom-domain-for-your-github-pages-site)."{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+* {% data variables.product.prodname_dotcom %} will no longer included the repository in the {% data variables.product.prodname_archive %}. For more information, see "[About archiving content and data on {% data variables.product.prodname_dotcom %}](/github/creating-cloning-and-archiving-repositories/about-archiving-content-and-data-on-github#about-the-github-archive-program)."{% endif %}{% if enterpriseServerVersions contains currentVersion %}
+* Anonymous Git read access is no longer available. For more information, see "[Enabling anonymous Git read access for a repository](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)."{% endif %}
- * {% data variables.product.prodname_dotcom %} will detach public forks of the public repository and put them into a new network. Öffentliche Forks werden nicht in private Forks umgewandelt. {% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %}If you change a repository's visibility from internal to private, {% data variables.product.prodname_dotcom %} will remove forks that belong to any user without access to the newly private repository.{% endif %} For more information, see "[What happens to forks when a repository is deleted or changes visibility?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility#changing-a-public-repository-to-a-private-repository)"
- {% if currentVersion == "free-pro-team@latest" %}* If you're using {% data variables.product.prodname_free_user %} for user accounts or organizations, some features won't be available in the repository after you change the visibility to private. {% data reusables.gated-features.more-info %}
- * Jede veröffentlichte {% data variables.product.prodname_pages %}-Website wird automatisch zurückgezogen. Wenn Sie Ihrer {% data variables.product.prodname_pages %}-Website eine benutzerdefinierte Domain hinzugefügt hatten, sollten Sie Ihre DNS-Einträge vor der Umschaltung des Repositorys in ein privates Repository entfernen oder aktualisieren, um das Risiko eines Domain-Takeovers auszuschließen. Weitere Informationen findest Du unter „[Eine benutzerdefinierte Domäne für Deine {% data variables.product.prodname_pages %}-Website verwalten](/articles/managing-a-custom-domain-for-your-github-pages-site).“
- * {% data variables.product.prodname_dotcom %} will no longer included the repository in the {% data variables.product.prodname_archive %}. For more information, see "[About archiving content and data on {% data variables.product.prodname_dotcom %}](/github/creating-cloning-and-archiving-repositories/about-archiving-content-and-data-on-github#about-the-github-archive-program)."{% endif %}
- {% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}* Anonymous Git read access is no longer available. Weitere Informationen finden Sie unter „[Anonymen Git-Lesezugriff für ein Repository aktivieren](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)“.{% endif %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %}
+
+#### Repository als intern festlegen
+
+{% note %}
+
+**Note:** {% data reusables.gated-features.internal-repos %}
+
+{% endnote %}
+
+* Any forks of the repository will remain in the repository network, and {% data variables.product.product_name %} maintains the relationship between the root repository and the fork. For more information, see "[What happens to forks when a repository is deleted or changes visibility?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility)"
+
+{% endif %}
+
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
#### Repository als öffentlich festlegen
- * {% data variables.product.prodname_dotcom %} will detach private forks and turn them into a standalone private repository. Weitere Informationen findest Du unter „[Was geschieht mit Forks, wenn ein Repository gelöscht wird oder sich dessen Sichtbarkeit ändert?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility#changing-a-private-repository-to-a-public-repository).“
- * If you're converting your private repository to a public repository as part of a move toward creating an open source project, see the [Open Source Guides](http://opensource.guide) for helpful tips and guidelines.{% if currentVersion == "free-pro-team@latest" %} You can also take a free course on managing an open source project with [{% data variables.product.prodname_learning %}]({% data variables.product.prodname_learning_link %}). Sobald Dein Repository der Öffentlichkeit zugänglich ist, kannst Du im Community-Profil des Repositorys überprüfen, ob Dein Projekt die Best Practices zur Unterstützung von Mitarbeitern erfüllt. Weitere Informationen finden Sie unter „[Community-Profil anzeigen](/articles/viewing-your-community-profile)“.{% endif %}
+* {% data variables.product.product_name %} will detach private forks and turn them into a standalone private repository. For more information, see "[What happens to forks when a repository is deleted or changes visibility?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility#changing-a-private-repository-to-a-public-repository)"{% if currentVersion == "free-pro-team@latest" %}
+* If you're converting your private repository to a public repository as part of a move toward creating an open source project, see the [Open Source Guides](http://opensource.guide) for helpful tips and guidelines. You can also take a free course on managing an open source project with [{% data variables.product.prodname_learning %}]({% data variables.product.prodname_learning_link %}). Sobald Dein Repository der Öffentlichkeit zugänglich ist, kannst Du im Community-Profil des Repositorys überprüfen, ob Dein Projekt die Best Practices zur Unterstützung von Mitarbeitern erfüllt. Weitere Informationen finden Sie unter „[Community-Profil anzeigen](/articles/viewing-your-community-profile)“.{% endif %}
+
+{% endif %}
{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
diff --git a/translations/de-DE/content/github/authenticating-to-github/about-anonymized-image-urls.md b/translations/de-DE/content/github/authenticating-to-github/about-anonymized-image-urls.md
index eb03fbcb3571..7e04fd221577 100644
--- a/translations/de-DE/content/github/authenticating-to-github/about-anonymized-image-urls.md
+++ b/translations/de-DE/content/github/authenticating-to-github/about-anonymized-image-urls.md
@@ -8,7 +8,7 @@ versions:
free-pro-team: '*'
---
-Zum Hosten Ihrer Bilder verwendet {% data variables.product.product_name %} den [Open-Source-Projekt-Camo](https://github.com/atmos/camo). Camo erzeugt für jedes Bild, das mit `https://camo.githubusercontent.com/` beginnt, einen anonymen URL-Proxy und verbirgt Deine Browserdaten und ähnliche Informationen vor anderen Benutzern.
+Zum Hosten Ihrer Bilder verwendet {% data variables.product.product_name %} den [Open-Source-Projekt-Camo](https://github.com/atmos/camo). Camo generates an anonymous URL proxy for each image which hides your browser details and related information from other users. The URL starts `https://.githubusercontent.com/`, with different subdomains depending on how you uploaded the image.
Jeder, der Deine anonymisierte Bild-URL direkt oder indirekt erhält, kann Dein Bild anzeigen. Beschränke vertrauliche Bilder zu deren Schutz auf ein privates Netzwerk oder einen Server, der eine Authentifizierung erfordert, anstatt Camo zu verwenden.
diff --git a/translations/de-DE/content/github/authenticating-to-github/adding-a-new-ssh-key-to-your-github-account.md b/translations/de-DE/content/github/authenticating-to-github/adding-a-new-ssh-key-to-your-github-account.md
index a5ca81da4a33..1e96f1d87aa9 100644
--- a/translations/de-DE/content/github/authenticating-to-github/adding-a-new-ssh-key-to-your-github-account.md
+++ b/translations/de-DE/content/github/authenticating-to-github/adding-a-new-ssh-key-to-your-github-account.md
@@ -24,8 +24,8 @@ Nachdem Sie einen neuen SSH-Schlüssel zu Ihrem {% data variables.product.produc
Wenn Deine SSH-Schlüsseldatei einen anderen Namen hat als die Datei im Beispielcode, passe den Dateinamen entsprechend an. Achte beim Kopieren des Schlüssels darauf, keine neuen Zeilen oder Leerzeichen hinzuzufügen.
```shell
- $ pbcopy < ~/.ssh/id_rsa.pub
- # Kopiert den Inhalt der Datei id_rsa.pub in die Zwischenablage
+ $ pbcopy < ~/.ssh/id_ed25519.pub
+ # Copies the contents of the id_ed25519.pub file to your clipboard
```
{% tip %}
@@ -51,8 +51,8 @@ Nachdem Sie einen neuen SSH-Schlüssel zu Ihrem {% data variables.product.produc
Wenn Deine SSH-Schlüsseldatei einen anderen Namen hat als die Datei im Beispielcode, passe den Dateinamen entsprechend an. Achte beim Kopieren des Schlüssels darauf, keine neuen Zeilen oder Leerzeichen hinzuzufügen.
```shell
- $ clip < ~/.ssh/id_rsa.pub
- # Kopiert den Inhalt der Datei id_rsa.pub in die Zwischenablage
+ $ clip < ~/.ssh/id_ed25519.pub
+ # Copies the contents of the id_ed25519.pub file to your clipboard
```
{% tip %}
@@ -81,8 +81,8 @@ Nachdem Sie einen neuen SSH-Schlüssel zu Ihrem {% data variables.product.produc
$ sudo apt-get install xclip
# Herunterladen und installieren von xclip. If you don't have `apt-get`, you might need to use another installer (like `yum`)
- $ xclip -selection clipboard < ~/.ssh/id_rsa.pub
- # Copies the contents of the id_rsa.pub file to your clipboard
+ $ xclip -selection clipboard < ~/.ssh/id_ed25519.pub
+ # Copies the contents of the id_ed25519.pub file to your clipboard
```
{% tip %}
diff --git a/translations/de-DE/content/github/authenticating-to-github/generating-a-new-ssh-key-and-adding-it-to-the-ssh-agent.md b/translations/de-DE/content/github/authenticating-to-github/generating-a-new-ssh-key-and-adding-it-to-the-ssh-agent.md
index aa137dc96208..52127b14d03c 100644
--- a/translations/de-DE/content/github/authenticating-to-github/generating-a-new-ssh-key-and-adding-it-to-the-ssh-agent.md
+++ b/translations/de-DE/content/github/authenticating-to-github/generating-a-new-ssh-key-and-adding-it-to-the-ssh-agent.md
@@ -98,13 +98,19 @@ Bevor Du einen neuen SSH-Schlüssel zum SSH-Agenten für die Verwaltung Deiner S
IdentityFile ~/.ssh/id_ed25519
```
+ {% note %}
+
+ **Note:** If you chose not to add a passphrase to your key, you should omit the `UseKeychain` line.
+
+ {% endnote %}
+
3. Fügen Sie Ihren privaten SSH-Schlüssel zu ssh-agent hinzu, und speichern Sie Ihre Passphrase in der Keychain. {% data reusables.ssh.add-ssh-key-to-ssh-agent %}
```shell
$ ssh-add -K ~/.ssh/id_ed25519
```
{% note %}
- **Hinweis:** Die Option `-K` ist die Standardversion von `ssh-add` von Apple, bei der die Passphrase für das Hinzufügen eines SSH-Schlüssels zum SSH-Agenten in Deiner Keychain gespeichert wird.
+ **Hinweis:** Die Option `-K` ist die Standardversion von `ssh-add` von Apple, bei der die Passphrase für das Hinzufügen eines SSH-Schlüssels zum SSH-Agenten in Deiner Keychain gespeichert wird. If you chose not to add a passphrase to your key, run the command without the `-K` option.
Wenn Sie die Standardversion von Apple nicht installiert haben, tritt möglicherweise ein Fehler auf. Weitere Informationen zum Beheben dieses Fehlers finden Sie unter [Fehler: „ssh-add: illegal option -- K“](/articles/error-ssh-add-illegal-option-k)“.
diff --git a/translations/de-DE/content/github/authenticating-to-github/reviewing-your-security-log.md b/translations/de-DE/content/github/authenticating-to-github/reviewing-your-security-log.md
index b7747cfe1405..f36a844efc86 100644
--- a/translations/de-DE/content/github/authenticating-to-github/reviewing-your-security-log.md
+++ b/translations/de-DE/content/github/authenticating-to-github/reviewing-your-security-log.md
@@ -1,6 +1,7 @@
---
title: Dein Sicherheitsprotokoll überprüfen
intro: Du kannst das Sicherheitsprotokoll für Dein Benutzerkonto überprüfen, um Dich betreffende Aktionen besser zu verstehen, die von Dir oder anderen Benutzern durchgeführt wurden.
+miniTocMaxHeadingLevel: 4
redirect_from:
- /articles/reviewing-your-security-log
versions:
@@ -30,216 +31,222 @@ The security log lists all actions performed within the last 90 days{% if curren
#### Suche nach der Art der durchgeführten Aktion
{% else %}
### Ereignisse im Sicherheitsprotokoll verstehen
+{% endif %}
+
+The events listed in your security log are triggered by your actions. Actions are grouped into the following categories:
+
+| Kategoriename | Beschreibung |
+| -------------------------------------------------------------------------------------------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |{% if currentVersion == "free-pro-team@latest" %}
+| [`account_recovery_token`](#account_recovery_token-category-actions) | Umfasst alle Aktivitäten in Verbindung mit dem [Hinzufügen eines Wiederherstellungstokens](/articles/configuring-two-factor-authentication-recovery-methods). |
+| [`Abrechnung`](#billing-category-actions) | Umfasst alle Aktivitäten in Verbindung mit Ihren Abrechnungsinformationen. |
+| [`marketplace_agreement_signature (Unterzeichnung Marketplace-Vereinbarung)`](#marketplace_agreement_signature-category-actions) | Umfasst alle Aktivitäten in Verbindung mit der Signierung der {% data variables.product.prodname_marketplace %}-Entwicklervereinbarung. |
+| [`marketplace_listing (Eintrag auf Marketplace)`](#marketplace_listing-category-actions) | Umfasst alle Aktivitäten in Verbindung mit dem Eintragen von Apps auf {% data variables.product.prodname_marketplace %}.{% endif %}
+| [`oauth_access`](#oauth_access-category-actions) | Contains all activities related to [{% data variables.product.prodname_oauth_app %}s](/articles/authorizing-oauth-apps) you've connected with.{% if currentVersion == "free-pro-team@latest" %}
+| [`payment_method`](#payment_method-category-actions) | Umfasst alle Aktivitäten in Verbindung mit der Bezahlung Ihres {% data variables.product.prodname_dotcom %}-Abonnements.{% endif %}
+| [`profile_picture`](#profile_picture-category-actions) | Umfasst alle Aktivitäten in Verbindung mit Ihrem Profilbild. |
+| [`project (Projekt)`](#project-category-actions) | Umfasst alle Aktivitäten in Verbindung mit Projektboards. |
+| [`public_key`](#public_key-category-actions) | Umfasst alle Aktivitäten in Verbindung mit [Ihren öffentlichen SSH-Schlüsseln](/articles/adding-a-new-ssh-key-to-your-github-account). |
+| [`repo`](#repo-category-actions) | Contains all activities related to the repositories you own.{% if currentVersion == "free-pro-team@latest" %}
+| [`sponsors`](#sponsors-category-actions) | Contains all events related to {% data variables.product.prodname_sponsors %} and sponsor buttons (see "[About {% data variables.product.prodname_sponsors %}](/articles/about-github-sponsors)" and "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)"){% endif %}{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
+| [`Team`](#team-category-actions) | Contains all activities related to teams you are a part of.{% endif %}{% if currentVersion != "github-ae@latest" %}
+| [`two_factor_authentication`](#two_factor_authentication-category-actions) | Contains all activities related to [two-factor authentication](/articles/securing-your-account-with-two-factor-authentication-2fa).{% endif %}
+| [`Benutzer`](#user-category-actions) | Umfasst alle Aktivitäten in Verbindung mit Deinem Konto. |
+
+{% if currentVersion == "free-pro-team@latest" %}
+
+### Dein Sicherheitsprotokoll exportieren
+
+{% data reusables.audit_log.export-log %}
+{% data reusables.audit_log.exported-log-keys-and-values %}
-Die Aktionen in Deinem Sicherheitsprotokoll sind nach folgenden Kategorien gruppiert:{% endif %}
-| Kategoriename | Beschreibung |
-| --------------------------------------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |{% if currentVersion == "free-pro-team@latest" %}
-| `account_recovery_token` | Umfasst alle Aktivitäten in Verbindung mit dem [Hinzufügen eines Wiederherstellungstokens](/articles/configuring-two-factor-authentication-recovery-methods). |
-| `Abrechnung` | Umfasst alle Aktivitäten in Verbindung mit Deinen Abrechnungsinformationen. |
-| `marketplace_agreement_signature (Unterzeichnung Marketplace-Vereinbarung)` | Umfasst alle Aktivitäten in Verbindung mit der Signierung der {% data variables.product.prodname_marketplace %}-Entwicklervereinbarung. |
-| `marketplace_listing (Eintrag auf Marketplace)` | Umfasst alle Aktivitäten in Verbindung mit dem Eintragen von Apps auf {% data variables.product.prodname_marketplace %}.{% endif %}
-| `oauth_access` | Contains all activities related to [{% data variables.product.prodname_oauth_app %}s](/articles/authorizing-oauth-apps) you've connected with.{% if currentVersion == "free-pro-team@latest" %}
-| `payment_method` | Umfasst alle Aktivitäten in Verbindung mit der Bezahlung Deines {% data variables.product.prodname_dotcom %}-Abonnements.{% endif %}
-| `profile_picture` | Umfasst alle Aktivitäten in Verbindung mit Deinem Profilbild. |
-| `project (Projekt)` | Umfasst alle Aktivitäten in Verbindung mit Projektboards. |
-| `public_key` | Umfasst alle Aktivitäten in Verbindung mit [Deinen öffentlichen SSH-Schlüsseln](/articles/adding-a-new-ssh-key-to-your-github-account). |
-| `repo` | Contains all activities related to the repositories you own.{% if currentVersion == "free-pro-team@latest" %}
-| `sponsors` | Contains all events related to {% data variables.product.prodname_sponsors %} and sponsor buttons (see "[About {% data variables.product.prodname_sponsors %}](/articles/about-github-sponsors)" and "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)"){% endif %}{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-| `Team` | Contains all activities related to teams you are a part of.{% endif %}{% if currentVersion != "github-ae@latest" %}
-| `two_factor_authentication` | Contains all activities related to [two-factor authentication](/articles/securing-your-account-with-two-factor-authentication-2fa).{% endif %}
-| `Benutzer` | Umfasst alle Aktivitäten in Verbindung mit Deinem Konto. |
-
-Eine Beschreibung der Ereignisse dieser Kategorien findest Du nachfolgend.
+{% endif %}
+
+### Security log actions
+
+An overview of some of the most common actions that are recorded as events in the security log.
{% if currentVersion == "free-pro-team@latest" %}
-#### Kategorie `account_recovery_token`
+#### `account_recovery_token` category actions
-| Aktion | Beschreibung |
-| ------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
-| confirm | Wird ausgelöst, wenn Du [ein neues Token erfolgreich bei einem Wiederherstellungsanbieter speicherst](/articles/configuring-two-factor-authentication-recovery-methods). |
-| recover | Wird ausgelöst, wenn Du [ein Kontowiederherstellungstoken erfolgreich einlöst](/articles/recovering-your-account-if-you-lose-your-2fa-credentials). |
-| recover_error | Wird ausgelöst, wenn ein Token verwendet wird, {% data variables.product.prodname_dotcom %} dieses aber nicht validieren kann. |
+| Aktion | Beschreibung |
+| --------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| `confirm` | Wird ausgelöst, wenn Du [ein neues Token erfolgreich bei einem Wiederherstellungsanbieter speicherst](/articles/configuring-two-factor-authentication-recovery-methods). |
+| `recover` | Wird ausgelöst, wenn Du [ein Kontowiederherstellungstoken erfolgreich einlöst](/articles/recovering-your-account-if-you-lose-your-2fa-credentials). |
+| `recover_error` | Wird ausgelöst, wenn ein Token verwendet wird, {% data variables.product.prodname_dotcom %} dieses aber nicht validieren kann. |
-#### Kategorie `billing` (Abrechnung)
+#### `billing` category actions
| Aktion | Beschreibung |
| --------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------ |
-| change_billing_type | Wird ausgelöst, wenn Sie Ihre [Zahlungsmethode](/articles/adding-or-editing-a-payment-method) für {% data variables.product.prodname_dotcom %} ändern. |
-| change_email | Wird ausgelöst, wenn Du [Deine E-Mail-Adresse änderst](/articles/changing-your-primary-email-address). |
+| `change_billing_type` | Wird ausgelöst, wenn Sie Ihre [Zahlungsmethode](/articles/adding-or-editing-a-payment-method) für {% data variables.product.prodname_dotcom %} ändern. |
+| `change_email` | Wird ausgelöst, wenn Du [Deine E-Mail-Adresse änderst](/articles/changing-your-primary-email-address). |
-#### Kategorie `marketplace_agreement_signature`
+#### `marketplace_agreement_signature` category actions
-| Aktion | Beschreibung |
-| ------ | --------------------------------------------------------------------------------------------------------------- |
-| create | Wird ausgelöst, wenn Du die {% data variables.product.prodname_marketplace %}-Entwicklervereinbarung signierst. |
+| Aktion | Beschreibung |
+| -------- | --------------------------------------------------------------------------------------------------------------- |
+| `create` | Wird ausgelöst, wenn Du die {% data variables.product.prodname_marketplace %}-Entwicklervereinbarung signierst. |
-#### Kategorie `marketplace_listing` (Eintrag auf Marketplace)
+#### `marketplace_listing` category actions
-| Aktion | Beschreibung |
-| ---------- | ----------------------------------------------------------------------------------------------------------------------------- |
-| genehmigen | Wird ausgelöst, wenn Dein Eintrag für die Aufnahme in {% data variables.product.prodname_marketplace %} genehmigt wird. |
-| create | Wird ausgelöst, wenn Du einen Eintrag für Deine App in {% data variables.product.prodname_marketplace %} erstellst. |
-| delist | Wird ausgelöst, wenn Ihr Listing von {% data variables.product.prodname_marketplace %} entfernt wird. |
-| redraft | Wird ausgelöst, wenn Dein Eintrag in den Entwurfsstatus zurückversetzt wird. |
-| reject | Wird ausgelöst, wenn Dein Eintrag für die Aufnahme in {% data variables.product.prodname_marketplace %} nicht genehmigt wird. |
+| Aktion | Beschreibung |
+| ------------ | ----------------------------------------------------------------------------------------------------------------------------- |
+| `genehmigen` | Wird ausgelöst, wenn Dein Eintrag für die Aufnahme in {% data variables.product.prodname_marketplace %} genehmigt wird. |
+| `create` | Wird ausgelöst, wenn Du einen Eintrag für Deine App in {% data variables.product.prodname_marketplace %} erstellst. |
+| `delist` | Wird ausgelöst, wenn Ihr Listing von {% data variables.product.prodname_marketplace %} entfernt wird. |
+| `redraft` | Wird ausgelöst, wenn Dein Eintrag in den Entwurfsstatus zurückversetzt wird. |
+| `reject` | Wird ausgelöst, wenn Dein Eintrag für die Aufnahme in {% data variables.product.prodname_marketplace %} nicht genehmigt wird. |
{% endif %}
-#### Kategorie `oauth_access`
+#### `oauth_access` category actions
-| Aktion | Beschreibung |
-| ------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| create | Wird ausgelöst, wenn Sie einer [{% data variables.product.prodname_oauth_app %} Zugriff erteilen](/articles/authorizing-oauth-apps). |
-| destroy | Wird ausgelöst, wenn Sie einer [{% data variables.product.prodname_oauth_app %} den Zugriff auf Ihr Konto entziehen](/articles/reviewing-your-authorized-integrations). |
+| Aktion | Beschreibung |
+| --------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `create` | Wird ausgelöst, wenn Sie einer [{% data variables.product.prodname_oauth_app %} Zugriff erteilen](/articles/authorizing-oauth-apps). |
+| `destroy` | Wird ausgelöst, wenn Sie einer [{% data variables.product.prodname_oauth_app %} den Zugriff auf Ihr Konto entziehen](/articles/reviewing-your-authorized-integrations). |
{% if currentVersion == "free-pro-team@latest" %}
-#### Kategorie `payment_method`
+#### `payment_method` category actions
-| Aktion | Beschreibung |
-| ------------- | ------------------------------------------------------------------------------------------------------------------- |
-| clear | Wird ausgelöst, wenn eine [registrierte Zahlungsmethode](/articles/removing-a-payment-method) entfernt wird. |
-| create | Wird ausgelöst, wenn eine Zahlungsmethode, beispielsweise eine Kreditkarte oder ein PayPal-Konto, hinzugefügt wird. |
-| aktualisieren | Wird ausgelöst, wenn eine vorhandene Zahlungsmethode geändert wird. |
+| Aktion | Beschreibung |
+| --------------- | ------------------------------------------------------------------------------------------------------------------- |
+| `clear` | Wird ausgelöst, wenn eine [registrierte Zahlungsmethode](/articles/removing-a-payment-method) entfernt wird. |
+| `create` | Wird ausgelöst, wenn eine Zahlungsmethode, beispielsweise eine Kreditkarte oder ein PayPal-Konto, hinzugefügt wird. |
+| `aktualisieren` | Wird ausgelöst, wenn eine vorhandene Zahlungsmethode geändert wird. |
{% endif %}
-#### Kategorie `profile_picture`
+#### `profile_picture` category actions
-| Aktion | Beschreibung |
-| ------------- | ---------------------------------------------------------------------------------------------------------- |
-| aktualisieren | Wird ausgelöst, wenn Du [Dein Profilbild festlegst oder änderst](/articles/setting-your-profile-picture/). |
+| Aktion | Beschreibung |
+| --------------- | ---------------------------------------------------------------------------------------------------------- |
+| `aktualisieren` | Wird ausgelöst, wenn Du [Dein Profilbild festlegst oder änderst](/articles/setting-your-profile-picture/). |
-#### Kategorie `project`
+#### `project` category actions
| Aktion | Beschreibung |
| ------------------------ | -------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `access` | Wird ausgelöst, wenn die Sichtbarkeit eines Projektboards geändert wird. |
| `create` | Wird bei der Erstellung eines Projektboards ausgelöst. |
| `rename` | Wird ausgelöst, wenn ein Projektboard umbenannt wird. |
| `aktualisieren` | Wird ausgelöst, wenn ein Projektboard geändert wird. |
| `delete` | Wird ausgelöst, wenn ein Projektboard gelöscht wird. |
| `link` | Wird ausgelöst, wenn ein Repository mit einem Projektboard verknüpft wird. |
| `unlink` | Wird ausgelöst, wenn die Verknüpfung eines Repositorys mit einem Projektboard aufgehoben wird. |
-| `project.access` | Wird ausgelöst, wenn die Sichtbarkeit eines Projektboards geändert wird. |
| `update_user_permission` | Wird ausgelöst, wenn ein externer Mitarbeiter einem Projektboard hinzugefügt oder entfernt wird oder wenn sich seine Berechtigungsebene verändert. |
-#### Kategorie `public_key`
+#### `public_key` category actions
-| Aktion | Beschreibung |
-| ------ | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
-| create | Wird ausgelöst, wenn Sie [Ihrem {% data variables.product.product_name %}-Konto einen neuen öffentlichen SSH-Schlüssel hinzufügen](/articles/adding-a-new-ssh-key-to-your-github-account). |
-| delete | Wird ausgelöst, wenn Sie [einen öffentlichen SSH-Schlüssel aus Ihrem {% data variables.product.product_name %}-Konto entfernen](/articles/reviewing-your-ssh-keys). |
+| Aktion | Beschreibung |
+| -------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| `create` | Wird ausgelöst, wenn Sie [Ihrem {% data variables.product.product_name %}-Konto einen neuen öffentlichen SSH-Schlüssel hinzufügen](/articles/adding-a-new-ssh-key-to-your-github-account). |
+| `delete` | Wird ausgelöst, wenn Sie [einen öffentlichen SSH-Schlüssel aus Ihrem {% data variables.product.product_name %}-Konto entfernen](/articles/reviewing-your-ssh-keys). |
-#### Kategorie `repo`
+#### `repo` category actions
| Aktion | Beschreibung |
| ------------------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| access | Wird ausgelöst, wenn die Sichtbarkeit eines Repositorys, dessen Inhaber Du bist, [von „privat“ auf „öffentlich“ gesetzt wird](/articles/making-a-private-repository-public) (oder umgekehrt). |
-| add_member | Triggered when a {% data variables.product.product_name %} user is {% if currentVersion == "free-pro-team@latest" %}[invited to have collaboration access](/articles/inviting-collaborators-to-a-personal-repository){% else %}[given collaboration access](/articles/inviting-collaborators-to-a-personal-repository){% endif %} to a repository. |
-| add_topic | Wird ausgelöst, wenn ein Repository-Inhaber einem Repository [ein Thema hinzufügt](/articles/classifying-your-repository-with-topics). |
-| archived | Triggered when a repository owner [archives a repository](/articles/about-archiving-repositories).{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-| config.disable_anonymous_git_access | Wird ausgelöst, wenn für ein öffentliches Repository der [anonyme Git-Lesezugriff deaktiviert](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository) wird. |
-| config.enable_anonymous_git_access | Wird ausgelöst, wenn für ein öffentliches Repository der [anonyme Git-Lesezugriff aktiviert](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository) wird. |
-| config.lock_anonymous_git_access | Wird ausgelöst, wenn für ein Repository die [Einstellung für den anonymen Git-Lesezugriff gesperrt](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access) wird. |
-| config.unlock_anonymous_git_access | Wird ausgelöst, wenn für ein Repository die [Einstellungssperre für den anonymen Git-Lesezugriff aufgehoben](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access) wird.{% endif %}
-| create | Wird ausgelöst, wenn [ein neues Repository erstellt](/articles/creating-a-new-repository) wird. |
-| destroy | Triggered when [a repository is deleted](/articles/deleting-a-repository).{% if currentVersion == "free-pro-team@latest" %}
-| deaktivieren | Triggered when a repository is disabled (e.g., for [insufficient funds](/articles/unlocking-a-locked-account)).{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-| aktivieren | Wird ausgelöst, wenn ein Repository wieder aktiviert wird.{% endif %}
-| remove_member | Wird ausgelöst, wenn ein {% data variables.product.product_name %}-Benutzer [als Mitarbeiter aus einem Repository entfernt wird](/articles/removing-a-collaborator-from-a-personal-repository). |
-| remove_topic | Wird ausgelöst, wenn ein Repository-Inhaber ein Thema aus einem Repository entfernt. |
-| rename | Wird ausgelöst, wenn ein [Repository umbenannt](/articles/renaming-a-repository) wird. |
-| übertragen | Wird ausgelöst, wenn ein [Repository übertragen](/articles/how-to-transfer-a-repository) wird. |
-| transfer_start | Wird ausgelöst, wenn die Übertragung eines Repositorys initiiert wurde. |
-| unarchived | Wird ausgelöst, wenn ein Repository-Inhaber die Archivierung eines Repositorys aufhebt. |
+| `access` | Wird ausgelöst, wenn die Sichtbarkeit eines Repositorys, dessen Inhaber Du bist, [von „privat“ auf „öffentlich“ gesetzt wird](/articles/making-a-private-repository-public) (oder umgekehrt). |
+| `add_member` | Triggered when a {% data variables.product.product_name %} user is {% if currentVersion == "free-pro-team@latest" %}[invited to have collaboration access](/articles/inviting-collaborators-to-a-personal-repository){% else %}[given collaboration access](/articles/inviting-collaborators-to-a-personal-repository){% endif %} to a repository. |
+| `add_topic` | Wird ausgelöst, wenn ein Repository-Inhaber einem Repository [ein Thema hinzufügt](/articles/classifying-your-repository-with-topics). |
+| `archived` | Wird ausgelöst, wenn ein Repository-Inhaber ein [Repository archiviert](/articles/about-archiving-repositories).{% if enterpriseServerVersions contains currentVersion %}
+| `config.disable_anonymous_git_access` | Wird ausgelöst, wenn für ein öffentliches Repository der [anonyme Git-Lesezugriff deaktiviert](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository) wird. |
+| `config.enable_anonymous_git_access` | Wird ausgelöst, wenn für ein öffentliches Repository der [anonyme Git-Lesezugriff aktiviert](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository) wird. |
+| `config.lock_anonymous_git_access` | Wird ausgelöst, wenn für ein Repository die [Einstellung für den anonymen Git-Lesezugriff gesperrt](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access) wird. |
+| `config.unlock_anonymous_git_access` | Wird ausgelöst, wenn für ein Repository die [Einstellungssperre für den anonymen Git-Lesezugriff aufgehoben](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access) wird.{% endif %}
+| `create` | Wird bei der [Erstellung eines neuen Repositorys](/articles/creating-a-new-repository) ausgelöst. |
+| `destroy` | Triggered when [a repository is deleted](/articles/deleting-a-repository).{% if currentVersion == "free-pro-team@latest" %}
+| `deaktivieren` | Triggered when a repository is disabled (e.g., for [insufficient funds](/articles/unlocking-a-locked-account)).{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| `aktivieren` | Wird ausgelöst, wenn ein Repository wieder aktiviert wird.{% endif %}
+| `remove_member` | Wird ausgelöst, wenn ein {% data variables.product.product_name %}-Benutzer [als Mitarbeiter aus einem Repository entfernt wird](/articles/removing-a-collaborator-from-a-personal-repository). |
+| `remove_topic` | Wird ausgelöst, wenn ein Repository-Inhaber ein Thema aus einem Repository entfernt. |
+| `rename` | Wird ausgelöst, wenn ein [Repository umbenannt](/articles/renaming-a-repository) wird. |
+| `übertragen` | Wird ausgelöst, wenn ein [Repository übertragen](/articles/how-to-transfer-a-repository) wird. |
+| `transfer_start` | Wird ausgelöst, wenn die Übertragung eines Repositorys initiiert wurde. |
+| `unarchived` | Wird ausgelöst, wenn ein Repository-Inhaber die Archivierung eines Repositorys aufhebt. |
{% if currentVersion == "free-pro-team@latest" %}
-#### Kategorie `sponsors`
-
-| Aktion | Beschreibung |
-| ----------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| repo_funding_link_button_toggle | Wird ausgelöst, wenn Du eine Sponsorenschaltfläche in Deinem Repository aktivierst oder deaktivierst (siehe „[Sponsorenschaltfläche in Deinem Repository anzeigen](/articles/displaying-a-sponsor-button-in-your-repository)“) |
-| repo_funding_links_file_action | Wird ausgelöst, wenn Du die FUNDING-Datei in Deinem Repository änderst (siehe „[Sponsorenschaltfläche in Deinem Repository anzeigen](/articles/displaying-a-sponsor-button-in-your-repository)“) |
-| sponsor_sponsorship_cancel | Wird ausgelöst, wenn Du ein Sponsoring beendest (siehe „[Sponsoring herabstufen](/articles/downgrading-a-sponsorship)“) |
-| sponsor_sponsorship_create | Wird ausgelöst, wenn Du einen Entwickler unterstützt (siehe „[Unterstützen eines Open-Source-Mitarbeiters](/github/supporting-the-open-source-community-with-github-sponsors/sponsoring-an-open-source-contributor#sponsoring-a-developer)") |
-| sponsor_sponsorship_preference_change | Wird ausgelöst, wenn Du Deine Einstellung zum Empfangen von E-Mail-Updates von einem unterstützten Entwickler änderst (siehe „[Dein Sponsoring verwalten](/articles/managing-your-sponsorship)“) |
-| sponsor_sponsorship_tier_change | Wird ausgelöst, wenn Du Dein Sponsoring herauf- oder herabstufst (siehe „[Sponsoring heraufstufen](/articles/upgrading-a-sponsorship)“ und „[Sponsoring herabstufen](/articles/downgrading-a-sponsorship)“) |
-| sponsored_developer_approve | Wird ausgelöst, wenn Dein {% data variables.product.prodname_sponsors %}-Konto genehmigt ist (siehe „[{% data variables.product.prodname_sponsors %} für Dein Benutzerkonto aufsetzen](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
-| sponsored_developer_create | Wird aufgelöst, wenn Dein {% data variables.product.prodname_sponsors %}-Konto erstellt wird (siehe „[{% data variables.product.prodname_sponsors %} für Dein Benutzerkonto aufsetzen](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
-| sponsored_developer_profile_update | Wird ausgelöst, wenn Du Dein „unterstützter Benutzer"-Profil veränderst (siehe „[Deine Profildetails für {% data variables.product.prodname_sponsors %} verändern](/github/supporting-the-open-source-community-with-github-sponsors/editing-your-profile-details-for-github-sponsors)") |
-| sponsored_developer_request_approval | Wird ausgelöst, wenn Du Deine Bewerbung für {% data variables.product.prodname_sponsors %} für die Bewilligung einreichst (siehe „[{% data variables.product.prodname_sponsors %} für Dein Benutzerkonto aufsetzen](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
-| sponsored_developer_tier_description_update | Wird ausgelöst, wenn Du die Beschreibung einer Sponsoring-Stufe änderst (siehe „[Sponsoring-Stufen ändern](/articles/changing-your-sponsorship-tiers)“) |
-| sponsored_developer_update_newsletter_send | Wird ausgelöst, wenn Du Deinen Sponsoren einen E-Mail-Update sendest (siehe „[Sponsoren kontaktieren](/articles/contacting-your-sponsors)“) |
-| waitlist_invite_sponsored_developer | Wird ausgelöst, wenn Du eingeladen wirst, {% data variables.product.prodname_sponsors %} von der Warteliste her beizutreten (siehe „[{% data variables.product.prodname_sponsors %} für Dein Benutzerkonto aufsetzen](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
-| waitlist_join | Wird ausgelöst, wenn Du der Warteliste beitrittst, um ein „unterstützter Entwickler" zu werden (siehe [{% data variables.product.prodname_sponsors %} für Dein Benutzerkonto aufsetzen](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
+#### `sponsors` category actions
+
+| Aktion | Beschreibung |
+| --------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `repo_funding_link_button_toggle` | Wird ausgelöst, wenn Du eine Sponsorenschaltfläche in Deinem Repository aktivierst oder deaktivierst (siehe „[Sponsorenschaltfläche in Deinem Repository anzeigen](/articles/displaying-a-sponsor-button-in-your-repository)“) |
+| `repo_funding_links_file_action` | Wird ausgelöst, wenn Du die FUNDING-Datei in Deinem Repository änderst (siehe „[Sponsorenschaltfläche in Deinem Repository anzeigen](/articles/displaying-a-sponsor-button-in-your-repository)“) |
+| `sponsor_sponsorship_cancel` | Wird ausgelöst, wenn Sie ein Sponsoring beenden (siehe „[Sponsoring herabstufen](/articles/downgrading-a-sponsorship)“). |
+| `sponsor_sponsorship_create` | Wird ausgelöst, wenn Du einen Entwickler unterstützt (siehe „[Unterstützen eines Open-Source-Mitarbeiters](/github/supporting-the-open-source-community-with-github-sponsors/sponsoring-an-open-source-contributor#sponsoring-a-developer)") |
+| `sponsor_sponsorship_preference_change` | Wird ausgelöst, wenn Sie Ihre Einstellung zum Empfangen von E-Mail-Aktualisierungen von einem gesponserten Entwickler ändern (siehe „[Sponsoring verwalten](/articles/managing-your-sponsorship)“). |
+| `sponsor_sponsorship_tier_change` | Wird ausgelöst, wenn Sie Ihr Sponsoring upgraden oder herabstufen (siehe „[Sponsoring upgraden](/articles/upgrading-a-sponsorship)“ und „[Sponsoring herabstufen](/articles/downgrading-a-sponsorship)“). |
+| `sponsored_developer_approve` | Wird ausgelöst, wenn Dein {% data variables.product.prodname_sponsors %}-Konto genehmigt ist (siehe „[{% data variables.product.prodname_sponsors %} für Dein Benutzerkonto aufsetzen](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
+| `sponsored_developer_create` | Wird aufgelöst, wenn Dein {% data variables.product.prodname_sponsors %}-Konto erstellt wird (siehe „[{% data variables.product.prodname_sponsors %} für Dein Benutzerkonto aufsetzen](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
+| `sponsored_developer_profile_update` | Wird ausgelöst, wenn Du Dein „unterstützter Benutzer"-Profil veränderst (siehe „[Deine Profildetails für {% data variables.product.prodname_sponsors %} verändern](/github/supporting-the-open-source-community-with-github-sponsors/editing-your-profile-details-for-github-sponsors)") |
+| `sponsored_developer_request_approval` | Wird ausgelöst, wenn Du Deine Bewerbung für {% data variables.product.prodname_sponsors %} für die Bewilligung einreichst (siehe „[{% data variables.product.prodname_sponsors %} für Dein Benutzerkonto aufsetzen](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
+| `sponsored_developer_tier_description_update` | Wird ausgelöst, wenn Sie die Beschreibung einer Sponsoring-Stufe ändern (siehe „[Sponsoring-Stufen ändern](/articles/changing-your-sponsorship-tiers)“). |
+| `sponsored_developer_update_newsletter_send` | Wird ausgelöst, wenn Sie Ihren Sponsoren eine E-Mail-Aktualisierung senden (siehe „[Sponsoren kontaktieren](/articles/contacting-your-sponsors)“). |
+| `waitlist_invite_sponsored_developer` | Wird ausgelöst, wenn Du eingeladen wirst, {% data variables.product.prodname_sponsors %} von der Warteliste her beizutreten (siehe „[{% data variables.product.prodname_sponsors %} für Dein Benutzerkonto aufsetzen](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
+| `waitlist_join` | Wird ausgelöst, wenn Du der Warteliste beitrittst, um ein „unterstützter Entwickler" zu werden (siehe [{% data variables.product.prodname_sponsors %} für Dein Benutzerkonto aufsetzen](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
{% endif %}
{% if currentVersion == "free-pro-team@latest" %}
-#### Kategorie `successor_invitation`
-
-| Aktion | Beschreibung |
-| ------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
-| accept | Wird ausgelöst, wenn du eine Erneuerungseinladung annimmst (siehe „[Inhaber-Kontinuität Deiner Benutzerkonto-Repositorys aufrechterhalten](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
-| cancel | Wird ausgelöst, wenn du eine Erneuerungseinladung kündigst (siehe „[Inhaber-Kontinuität Deiner Benutzerkonto-Repositorys aufrechterhalten](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
-| create | Wird ausgelöst, wenn du eine Erneuerungseinladung erstellst (siehe „[Inhaber-Kontinuität Deiner Benutzerkonto-Repositorys aufrechterhalten](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
-| decline | Wird ausgelöst, wenn du eine Erneuerungseinladung ablehnst (siehe „[Inhaber-Kontinuität Deiner Benutzerkonto-Repositorys aufrechterhalten](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
-| revoke | Wird ausgelöst, wenn du eine Erneuerungseinladung zurückziehst (siehe „[Inhaber-Kontinuität Deiner Benutzerkonto-Repositorys aufrechterhalten](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
+#### `successor_invitation` category actions
+
+| Aktion | Beschreibung |
+| --------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| `accept` | Wird ausgelöst, wenn du eine Erneuerungseinladung annimmst (siehe „[Inhaber-Kontinuität Deiner Benutzerkonto-Repositorys aufrechterhalten](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
+| `cancel` | Wird ausgelöst, wenn du eine Erneuerungseinladung kündigst (siehe „[Inhaber-Kontinuität Deiner Benutzerkonto-Repositorys aufrechterhalten](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
+| `create` | Wird ausgelöst, wenn du eine Erneuerungseinladung erstellst (siehe „[Inhaber-Kontinuität Deiner Benutzerkonto-Repositorys aufrechterhalten](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
+| `decline` | Wird ausgelöst, wenn du eine Erneuerungseinladung ablehnst (siehe „[Inhaber-Kontinuität Deiner Benutzerkonto-Repositorys aufrechterhalten](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
+| `revoke` | Wird ausgelöst, wenn du eine Erneuerungseinladung zurückziehst (siehe „[Inhaber-Kontinuität Deiner Benutzerkonto-Repositorys aufrechterhalten](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
{% endif %}
{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-#### Kategorie `team`
+#### `team` category actions
-| Aktion | Beschreibung |
-| ----------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
-| add_member | Wird ausgelöst, wenn ein Mitglied einer Organisation, zu der Du gehörst, [Dich zu einem Team hinzufügt](/articles/adding-organization-members-to-a-team). |
-| add_repository | Wird ausgelöst, wenn ein Team, dessen Mitglied Du bist, die Kontrolle über ein Repository erhält. |
-| create | Wird ausgelöst, wenn in einer Organisation, zu der Du gehörst, ein neues Team erstellt wird. |
-| destroy | Wird ausgelöst, wenn ein Team, dessen Mitglied Du bist, aus einer Organisation gelöscht wird. |
-| remove_member | Wird ausgelöst, wenn ein Mitglied einer Organisation [aus einem Team entfernt wird](/articles/removing-organization-members-from-a-team), dessen Mitglied Du bist. |
-| remove_repository | Wird ausgelöst, wenn ein Repository nicht mehr unter der Kontrolle eines Teams steht. |
+| Aktion | Beschreibung |
+| ------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `add_member` | Wird ausgelöst, wenn ein Mitglied einer Organisation, zu der Sie gehören, [Sie zu einem Team hinzufügt](/articles/adding-organization-members-to-a-team). |
+| `add_repository` | Wird ausgelöst, wenn ein Team, dessen Mitglied Sie sind, die Kontrolle über ein Repository erhält. |
+| `create` | Wird ausgelöst, wenn in einer Organisation, zu der Sie gehören, ein neues Team erstellt wird. |
+| `destroy` | Wird ausgelöst, wenn ein Team, dessen Mitglied Sie sind, aus einer Organisation gelöscht wird. |
+| `remove_member` | Wird ausgelöst, wenn ein Mitglied einer Organisation aus einem [Team entfernt wird](/articles/removing-organization-members-from-a-team), dessen Mitglied Sie sind. |
+| `remove_repository` | Wird ausgelöst, wenn ein Repository nicht mehr unter der Kontrolle eines Teams steht. |
{% endif %}
{% if currentVersion != "github-ae@latest" %}
-#### Kategorie `two_factor_authentication`
+#### `two_factor_authentication` category actions
-| Aktion | Beschreibung |
-| -------- | ------------------------------------------------------------------------------------------------------------------------------------------- |
-| enabled | Wird bei der Aktivierung der [Zwei-Faktor-Authentifizierung](/articles/securing-your-account-with-two-factor-authentication-2fa) ausgelöst. |
-| disabled | Wird bei der Deaktivierung der Zwei-Faktor-Authentifizierung ausgelöst. |
+| Aktion | Beschreibung |
+| ---------- | ------------------------------------------------------------------------------------------------------------------------------------------- |
+| `enabled` | Wird bei der Aktivierung der [Zwei-Faktor-Authentifizierung](/articles/securing-your-account-with-two-factor-authentication-2fa) ausgelöst. |
+| `disabled` | Wird bei der Deaktivierung der Zwei-Faktor-Authentifizierung ausgelöst. |
{% endif %}
-#### Kategorie `user`
-
-| Aktion | Beschreibung |
-| ---------------------------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| add_email | Triggered when you {% if currentVersion != "github-ae@latest" %}[add a new email address](/articles/changing-your-primary-email-address){% else %}add a new email address{% endif %}. |
-| create | Wird ausgelöst, wenn Sie ein neues Benutzerkonto erstellen. |
-| remove_email | Wird ausgelöst, wenn Sie eine E-Mail-Adresse entfernen. |
-| rename | Triggered when you rename your account.{% if currentVersion != "github-ae@latest" %}
-| change_password | Wird ausgelöst, wenn Sie Ihr Passwort ändern. |
-| forgot_password | Triggered when you ask for [a password reset](/articles/how-can-i-reset-my-password).{% endif %}
-| login | Wird ausgelöst, wenn Du Dich bei {% data variables.product.product_location %} anmeldest. |
-| failed_login | Triggered when you failed to log in successfully.{% if currentVersion != "github-ae@latest" %}
-| two_factor_requested | Triggered when {% data variables.product.product_name %} asks you for [your two-factor authentication code](/articles/accessing-github-using-two-factor-authentication).{% endif %}
-| show_private_contributions_count | Wird ausgelöst, wenn Sie [private Beiträge in Ihrem Profil veröffentlichen](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile). |
-| hide_private_contributions_count | Triggered when you [hide private contributions on your profile](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile).{% if currentVersion == "free-pro-team@latest" %}
-| report_content | Wird ausgelöst, wenn Sie [ein Issue oder einen Pull Request bzw. einen Kommentar zu einem Issue, einem Pull Request oder einem Commit melden](/articles/reporting-abuse-or-spam).{% endif %}
-
-#### Kategorie `user_status`
-
-| Aktion | Beschreibung |
-| ------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| aktualisieren | Wird ausgelöst, wenn Sie den Status Ihres Profils festlegen oder ändern. Weitere Informationen findest Du unter „[Status festlegen](/articles/personalizing-your-profile/#setting-a-status).“ |
-| destroy | Wird ausgelöst, wenn Sie den Status Ihres Profils löschen. |
+#### `user` category actions
-{% if currentVersion == "free-pro-team@latest" %}
+| Aktion | Beschreibung |
+| ---------------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `add_email` | Triggered when you {% if currentVersion != "github-ae@latest" %}[add a new email address](/articles/changing-your-primary-email-address){% else %}add a new email address{% endif %}. |
+| `create` | Triggered when you create a new user account.{% if currentVersion != "github-ae@latest" %}
+| `change_password` | Wird ausgelöst, wenn Sie Ihr Passwort ändern. |
+| `forgot_password` | Triggered when you ask for [a password reset](/articles/how-can-i-reset-my-password).{% endif %}
+| `hide_private_contributions_count` | Wird ausgelöst, wenn Sie [private Beiträge in Ihrem Profil verbergen](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile). |
+| `login` | Wird ausgelöst, wenn Du Dich bei {% data variables.product.product_location %} anmeldest. |
+| `failed_login` | Wird ausgelöst, wenn Ihre Anmeldung fehlschlägt. |
+| `remove_email` | Wird ausgelöst, wenn Sie eine E-Mail-Adresse entfernen. |
+| `rename` | Triggered when you rename your account.{% if currentVersion == "free-pro-team@latest" %}
+| `report_content` | Wird ausgelöst, wenn Sie [ein Issue oder einen Pull Request bzw. einen Kommentar zu einem Issue, einem Pull Request oder einem Commit melden](/articles/reporting-abuse-or-spam).{% endif %}
+| `show_private_contributions_count` | Triggered when you [publicize private contributions on your profile](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile).{% if currentVersion != "github-ae@latest" %}
+| `two_factor_requested` | Triggered when {% data variables.product.product_name %} asks you for [your two-factor authentication code](/articles/accessing-github-using-two-factor-authentication).{% endif %}
-### Dein Sicherheitsprotokoll exportieren
+#### `user_status` category actions
+
+| Aktion | Beschreibung |
+| --------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `aktualisieren` | Wird ausgelöst, wenn Du den Status Deines Profils festlegst oder änderst. Weitere Informationen findest Du unter „[Status festlegen](/articles/personalizing-your-profile/#setting-a-status).“ |
+| `destroy` | Wird ausgelöst, wenn Du den Status Deines Profils löschst. |
-{% data reusables.audit_log.export-log %}
-{% data reusables.audit_log.exported-log-keys-and-values %}
-{% endif %}
diff --git a/translations/de-DE/content/github/building-a-strong-community/about-issue-and-pull-request-templates.md b/translations/de-DE/content/github/building-a-strong-community/about-issue-and-pull-request-templates.md
index 9f8726532305..d77c34c95a03 100644
--- a/translations/de-DE/content/github/building-a-strong-community/about-issue-and-pull-request-templates.md
+++ b/translations/de-DE/content/github/building-a-strong-community/about-issue-and-pull-request-templates.md
@@ -11,7 +11,11 @@ versions:
Nachdem Du in Deinem Repository Vorlagen für Issues und Pull Requests erstellt hast, können Mitarbeiter die Vorlagen verwenden, um Issues zu öffnen oder vorgeschlagene Änderungen in ihren Pull Requests gemäß den Beitragsrichtlinien des Repositorys zu beschreiben. Weitere Informationen zum Hinzufügen von Beitragsrichtlinien zu einem Repository findest Du unter „[Richtlinien für Repository-Mitarbeiter festlegen](/articles/setting-guidelines-for-repository-contributors).“
-You can create default issue and pull request templates for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. Weitere Informationen findest Du unter „[Eine Standard Community-Unterstützungsdatei erstellen](/github/building-a-strong-community/creating-a-default-community-health-file)."
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+You can create default issue and pull request templates for your organization or user account. Weitere Informationen findest Du unter „[Eine Standard Community-Unterstützungsdatei erstellen](/github/building-a-strong-community/creating-a-default-community-health-file)."
+
+{% endif %}
### Vorlagen für Issues
@@ -25,9 +29,9 @@ Mit dem Vorlagengenerator kannst Du für jede Vorlage einen Titel und eine Besch
{% data reusables.repositories.issue-template-config %} Weitere Informationen findest Du unter „[Issuevorlagen für Dein Repository konfigurieren](/github/building-a-strong-community/configuring-issue-templates-for-your-repository#configuring-the-template-chooser)."
{% endif %}
-Issuevorlagen werden auf dem Standardbranch des Repositorys in einem verborgenen `.github/ISSUE_TEMPLATE`-Verzeichnis gespeichert. Wenn Du eine Vorlage in einem anderen Branch erstellst, steht sie Mitarbeitern nicht zur Verfügung. Bei den Dateinamen von Issuevorlagen wird nicht zwischen Groß- und Kleinschreibung unterschieden, und es wird eine *.md*-Erweiterung benötigt. {% data reusables.repositories.valid-community-issues %}
+Issue-Vorlagen werden auf dem Standardbranch des Repositorys in einem verborgenen `.github/ISSUE_TEMPLATE`-Verzeichnis gespeichert. Wenn Sie eine Vorlage in einem anderen Branch erstellen, steht sie Mitarbeitern nicht zur Verfügung. Bei den Dateinamen von Issuevorlagen wird nicht zwischen Groß- und Kleinschreibung unterschieden, und es wird eine *.md*-Erweiterung benötigt. {% data reusables.repositories.valid-community-issues %}
-Es ist möglich, manuell eine einzelne Issuevorlage in Markdown mit dem Workflow für ältere Issuevorlagen zu erstellen, und Projektmitarbeiter werden automatisch den Inhalt der Vorlage im Issue-Text sehen. Wir empfehlen jedoch, den aktualisierten Mehrfach-Issue-Vorlagengenerator zu verwenden, um Issuevorlagen zu erstellen. Weitere Informationen zum veralteten Workflow findest Du unter „[Eine einzelne Issue-Vorlage für Dein Repository manuell erstellen](/articles/manually-creating-a-single-issue-template-for-your-repository).“
+Es ist möglich, manuell eine einzelne Issue-Vorlage in Markdown mit dem Workflow für ältere Issue-Vorlagen zu erstellen, wobei die Projektmitarbeiter automatisch den Inhalt der Vorlage im Issue-Text sehen. Wir empfehlen jedoch, den aktualisierten Vorlagengenerator zum Erstellen mehrerer Issues zu verwenden, um Issue-Vorlagen zu erstellen. Weitere Informationen zum veralteten Workflow finden Sie unter „[Eine einzelne Issue-Vorlage für Ihr Repository manuell erstellen](/articles/manually-creating-a-single-issue-template-for-your-repository)“.
{% data reusables.repositories.security-guidelines %}
@@ -37,6 +41,6 @@ Wenn Sie eine Pull-Request-Vorlage zu Ihrem Repository hinzufügen, sehen Projek

-Du musst Vorlagen auf dem Standardbranch des Repositorys erstellen. Vorlagen, die in anderen Branches erstellt wurden, stehen Mitarbeitern nicht zur Verfügung. Du kannst Deine Vorlage für Pull Requests im sichtbaren Stammverzeichnis des Repositorys, im Ordner `docs` oder im verborgenen Verzeichnis `.github` speichern. Bei den Dateinamen von Pull-Request-Vorlagen wird nicht zwischen Groß- und Kleinschreibung unterschieden, und es kann eine *.md*- oder *.txt*-Erweiterung angefügt werden.
+Vorlagen müssen Sie auf dem Standardbranch des Repositorys erstellen. Vorlagen, die in anderen Branches erstellt wurden, stehen Mitarbeitern nicht zur Verfügung. Sie können Ihre Vorlage für Pull Requests im sichtbaren Stammverzeichnis des Repositorys, im Ordner `docs` oder im verborgenen Verzeichnis `.github` speichern. Bei den Dateinamen von Pull-Request-Vorlagen wird nicht zwischen Groß- und Kleinschreibung unterschieden, und es kann eine *.md*- oder *.txt*-Erweiterung angefügt werden.
-Weitere Informationen findest Du unter „[Eine Pull-Request-Vorlage für Dein Repository erstellen](/articles/creating-a-pull-request-template-for-your-repository).“
+Weitere Informationen finden Sie unter „[Eine Pull-Request-Vorlage für Ihr Repository erstellen](/articles/creating-a-pull-request-template-for-your-repository)“.
diff --git a/translations/de-DE/content/github/building-a-strong-community/about-wikis.md b/translations/de-DE/content/github/building-a-strong-community/about-wikis.md
index 048f5a28cf2a..71d2bda6d91c 100644
--- a/translations/de-DE/content/github/building-a-strong-community/about-wikis.md
+++ b/translations/de-DE/content/github/building-a-strong-community/about-wikis.md
@@ -15,9 +15,9 @@ Jedes {% data variables.product.product_name %}-Repository enthält einen Abschn
Mit Wikis können Sie Inhalte wie überall sonst auf {% data variables.product.product_name %} verfassen. Weitere Informationen findest Du unter „[Erste Schritte zum Schreiben und Formatieren auf {% data variables.product.prodname_dotcom %}](/articles/getting-started-with-writing-and-formatting-on-github)“. Wir verwenden [unsere Open-Source Markup-Bibliothek](https://github.com/github/markup), um verschiedene Formate in HTML zu konvertieren, sodass Du entscheiden kannst, in Markdown oder jedem anderen unterstützten Format zu schreiben.
-Wikis sind in öffentlichen Repositorys für die Öffentlichkeit zugänglich und in privaten Repositorys auf Personen mit Zugriff auf das Repository beschränkt. Weitere Informationen findest Du unter „[Sichtbarkeit eines Repositorys festlegen](/articles/setting-repository-visibility).“
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}If you create a wiki in a public repository, the wiki is available to {% if enterpriseServerVersions contains currentVersion %}anyone with access to {% data variables.product.product_location %}{% else %}the public{% endif %}. {% endif %}If you create a wiki in an internal or private repository, {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}people{% elsif currentVersion == "github-ae@latest" %}enterprise members{% endif %} with access to the repository can also access the wiki. Weitere Informationen findest Du unter „[Sichtbarkeit eines Repositorys festlegen](/articles/setting-repository-visibility).“
-Sie können Wikis direkt auf {% data variables.product.product_name %} bearbeiten, oder Sie können Wiki-Dateien lokal bearbeiten. Standardmäßig können nur Personen mit Schreibzugriff auf Ihr Repository Änderungen an Wikis vornehmen, jedoch können Sie jedem auf {% data variables.product.product_name %} erlauben, an einem Wiki in einem öffentlichen Repository mitzuwirken. Weitere Informationen findest Du unter „[Zugriffsberechtigungen für Wikis ändern](/articles/changing-access-permissions-for-wikis)“.
+Sie können Wikis direkt auf {% data variables.product.product_name %} bearbeiten, oder Sie können Wiki-Dateien lokal bearbeiten. By default, only people with write access to your repository can make changes to wikis, although you can allow everyone on {% data variables.product.product_location %} to contribute to a wiki in {% if currentVersion == "github-ae@latest" %}an internal{% else %}a public{% endif %} repository. Weitere Informationen findest Du unter „[Zugriffsberechtigungen für Wikis ändern](/articles/changing-access-permissions-for-wikis)“.
### Weiterführende Informationen
diff --git a/translations/de-DE/content/github/building-a-strong-community/adding-a-license-to-a-repository.md b/translations/de-DE/content/github/building-a-strong-community/adding-a-license-to-a-repository.md
index 4c4bfe1fff68..eb07b67f418e 100644
--- a/translations/de-DE/content/github/building-a-strong-community/adding-a-license-to-a-repository.md
+++ b/translations/de-DE/content/github/building-a-strong-community/adding-a-license-to-a-repository.md
@@ -6,7 +6,6 @@ redirect_from:
versions:
free-pro-team: '*'
enterprise-server: '*'
- github-ae: '*'
---
Wenn Du eine nachweisbare Lizenz in Dein Repository einfügst, wird sie den Benutzern, die Dein Repository besuchen, oben auf der Repository-Seite angezeigt. Um die gesamte Lizenzdatei zu lesen, klicke auf den Namen der Lizenz.
diff --git a/translations/de-DE/content/github/building-a-strong-community/adding-support-resources-to-your-project.md b/translations/de-DE/content/github/building-a-strong-community/adding-support-resources-to-your-project.md
index 027e2d3f1b44..d0f8dd8f7d04 100644
--- a/translations/de-DE/content/github/building-a-strong-community/adding-support-resources-to-your-project.md
+++ b/translations/de-DE/content/github/building-a-strong-community/adding-support-resources-to-your-project.md
@@ -13,7 +13,11 @@ Um Personen auf bestimmte Support-Ressourcen zu verweisen, kannst Du eine SUPPOR

-You can create default support resources for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. Weitere Informationen findest Du unter „[Eine Standard Community-Unterstützungsdatei erstellen](/github/building-a-strong-community/creating-a-default-community-health-file)."
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+You can create default support resources for your organization or user account. Weitere Informationen findest Du unter „[Eine Standard Community-Unterstützungsdatei erstellen](/github/building-a-strong-community/creating-a-default-community-health-file)."
+
+{% endif %}
{% tip %}
diff --git a/translations/de-DE/content/github/building-a-strong-community/configuring-issue-templates-for-your-repository.md b/translations/de-DE/content/github/building-a-strong-community/configuring-issue-templates-for-your-repository.md
index 4a181cca802f..f71b6880e9f1 100644
--- a/translations/de-DE/content/github/building-a-strong-community/configuring-issue-templates-for-your-repository.md
+++ b/translations/de-DE/content/github/building-a-strong-community/configuring-issue-templates-for-your-repository.md
@@ -10,10 +10,16 @@ versions:
github-ae: '*'
---
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
{% data reusables.repositories.default-issue-templates %}
+{% endif %}
+
{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %}
+
### Issuevorlagen erstellen
+
{% endif %}
{% data reusables.repositories.navigate-to-repo %}
diff --git a/translations/de-DE/content/github/building-a-strong-community/creating-a-default-community-health-file.md b/translations/de-DE/content/github/building-a-strong-community/creating-a-default-community-health-file.md
index 06b3f516b803..9737715ba8b9 100644
--- a/translations/de-DE/content/github/building-a-strong-community/creating-a-default-community-health-file.md
+++ b/translations/de-DE/content/github/building-a-strong-community/creating-a-default-community-health-file.md
@@ -7,7 +7,6 @@ redirect_from:
versions:
free-pro-team: '*'
enterprise-server: '*'
- github-ae: '*'
---
### Informationen zu Standard-Community-Unterstützungsdateien
diff --git a/translations/de-DE/content/github/building-a-strong-community/creating-a-pull-request-template-for-your-repository.md b/translations/de-DE/content/github/building-a-strong-community/creating-a-pull-request-template-for-your-repository.md
index fc5cbf961155..59cab3290ee0 100644
--- a/translations/de-DE/content/github/building-a-strong-community/creating-a-pull-request-template-for-your-repository.md
+++ b/translations/de-DE/content/github/building-a-strong-community/creating-a-pull-request-template-for-your-repository.md
@@ -13,7 +13,11 @@ Weitere Informationen findest Du unter „[Informationen zu Vorlagen für Issues
Du kannst in einem der unterstützten Ordner ein Unterverzeichnis *PULL_REQUEST_TEMPLATE* erstellen, um mehrere Pull-Request-Vorlagen zu speichern. Mit dem Abfrageparameter `template` kannst Du die Vorlage wählen, mit der der Pull-Request-Text ausgefüllt werden soll. Weitere Informationen findest Du unter „[Informationen zur Automatisierung für Issues und Pull Requests mit Abfrageparametern](/articles/about-automation-for-issues-and-pull-requests-with-query-parameters).“
-You can create default pull request templates for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. Weitere Informationen findest Du unter „[Eine Standard Community-Unterstützungsdatei erstellen](/github/building-a-strong-community/creating-a-default-community-health-file)."
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+You can create default pull request templates for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. Weitere Informationen findest Du unter „[Eine Standard Community-Unterstützungsdatei erstellen](/github/building-a-strong-community/creating-a-default-community-health-file)."
+
+{% endif %}
### Eine Pull-Request-Vorlage hinzufügen
diff --git a/translations/de-DE/content/github/building-a-strong-community/locking-conversations.md b/translations/de-DE/content/github/building-a-strong-community/locking-conversations.md
index e5ab101bde3f..7fe782c92950 100644
--- a/translations/de-DE/content/github/building-a-strong-community/locking-conversations.md
+++ b/translations/de-DE/content/github/building-a-strong-community/locking-conversations.md
@@ -15,7 +15,7 @@ Das Sperren einer Unterhaltung erstellt ein Zeitleistenereignis, das für alle B

-Solange eine Unterhaltung gesperrt ist, können nur [Personen mit Schreibzugriff](/articles/repository-permission-levels-for-an-organization/) und [Repository-Inhaber und -Mitarbeiter](/articles/permission-levels-for-a-user-account-repository/#collaborator-access-on-a-repository-owned-by-a-user-account) Kommentare hinzufügen, ausblenden und löschen.
+Solange eine Unterhaltung gesperrt ist, können nur [Personen mit Schreibzugriff](/articles/repository-permission-levels-for-an-organization/) und [Repository-Inhaber und -Mitarbeiter](/articles/permission-levels-for-a-user-account-repository/#collaborator-access-for-a-repository-owned-by-a-user-account) Kommentare hinzufügen, ausblenden und löschen.
Um nach gesperrten Unterhaltungen in einem nicht archivierten Repository zu suchen, kannst Du die Qualifizierer `is:locked` und `archived:false` verwenden. In archivierten Repositorys sind Unterhaltungen automatisch gesperrt. Weitere Informationen findest Du unter „[Issues und Pull Requests durchsuchen](/articles/searching-issues-and-pull-requests#search-based-on-whether-a-conversation-is-locked).“
diff --git a/translations/de-DE/content/github/building-a-strong-community/manually-creating-a-single-issue-template-for-your-repository.md b/translations/de-DE/content/github/building-a-strong-community/manually-creating-a-single-issue-template-for-your-repository.md
index ebd1bbade434..8123d5818735 100644
--- a/translations/de-DE/content/github/building-a-strong-community/manually-creating-a-single-issue-template-for-your-repository.md
+++ b/translations/de-DE/content/github/building-a-strong-community/manually-creating-a-single-issue-template-for-your-repository.md
@@ -39,8 +39,12 @@ assignees: octocat
{% endif %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
{% data reusables.repositories.default-issue-templates %}
+{% endif %}
+
### Eine Issuevorlage hinzufügen
{% data reusables.repositories.navigate-to-repo %}
diff --git a/translations/de-DE/content/github/building-a-strong-community/setting-guidelines-for-repository-contributors.md b/translations/de-DE/content/github/building-a-strong-community/setting-guidelines-for-repository-contributors.md
index 05dbfc1f8ea8..fbaffd61cbf4 100644
--- a/translations/de-DE/content/github/building-a-strong-community/setting-guidelines-for-repository-contributors.md
+++ b/translations/de-DE/content/github/building-a-strong-community/setting-guidelines-for-repository-contributors.md
@@ -20,7 +20,11 @@ Mitarbeitern helfen die Richtlinien, korrekt formulierte Pull Requests einzureic
Sowohl Inhaber als auch Mitarbeiter sparen dank Beitragsrichtlinien Zeit und Mühen, die durch fehlerhaft formulierte Pull Requests oder Issues entstehen, die abgelehnt und erneut eingereicht werden müssen.
-You can create default contribution guidelines for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. Weitere Informationen findest Du unter „[Eine Standard Community-Unterstützungsdatei erstellen](/github/building-a-strong-community/creating-a-default-community-health-file)."
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+You can create default contribution guidelines for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. Weitere Informationen findest Du unter „[Eine Standard Community-Unterstützungsdatei erstellen](/github/building-a-strong-community/creating-a-default-community-health-file)."
+
+{% endif %}
{% tip %}
@@ -45,7 +49,7 @@ You can create default contribution guidelines for your organization{% if curren
### Beispiele für Beitragsrichtlinien
-Wenn Du nun nicht sicher bist, was Du hier festlegen sollst, findest Du nachfolgend einige gute Beispiele für Beitragsrichtlinien:
+Wenn Sie nun nicht wissen, was Sie hier festlegen sollen, finden Sie nachfolgend einige gute Beispiele für Beitragsrichtlinien:
- [Beitragsrichtlinien](https://github.com/atom/atom/blob/master/CONTRIBUTING.md) für den Editor Atom
- [Beitragsrichtlinien](https://github.com/rails/rails/blob/master/CONTRIBUTING.md) für Ruby on Rails
@@ -53,5 +57,5 @@ Wenn Du nun nicht sicher bist, was Du hier festlegen sollst, findest Du nachfolg
### Weiterführende Informationen
- The Open Source Guides' section "[Starting an Open Source Project](https://opensource.guide/starting-a-project/)"{% if currentVersion == "free-pro-team@latest" %}
-- [{% data variables.product.prodname_learning %}]({% data variables.product.prodname_learning_link %}){% endif %}
-- „[Eine Lizenz zu einem Repository hinzufügen](/articles/adding-a-license-to-a-repository)“
+- [{% data variables.product.prodname_learning %}]({% data variables.product.prodname_learning_link %}){% endif %}{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- "[Adding a license to a repository](/articles/adding-a-license-to-a-repository)"{% endif %}
diff --git a/translations/de-DE/content/github/collaborating-with-issues-and-pull-requests/about-branches.md b/translations/de-DE/content/github/collaborating-with-issues-and-pull-requests/about-branches.md
index a4a405ba32ad..24a0da89bf53 100644
--- a/translations/de-DE/content/github/collaborating-with-issues-and-pull-requests/about-branches.md
+++ b/translations/de-DE/content/github/collaborating-with-issues-and-pull-requests/about-branches.md
@@ -23,7 +23,7 @@ Du benötigst Schreibzugriff auf ein Repository, um einen Branch zu erstellen, e
### About the default branch
-{% data reusables.branches.new-repo-default-branch %} The default branch is the branch that {% data variables.product.prodname_dotcom %} displays when anyone visits your repository. The default branch is also the initial branch that Git checks out locally out when someone clones the repository. {% data reusables.branches.default-branch-automatically-base-branch %}
+{% data reusables.branches.new-repo-default-branch %} The default branch is the branch that {% data variables.product.prodname_dotcom %} displays when anyone visits your repository. The default branch is also the initial branch that Git checks out locally when someone clones the repository. {% data reusables.branches.default-branch-automatically-base-branch %}
By default, {% data variables.product.product_name %} names the default branch {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}`main`{% else %}`master`{% endif %} in any new repository.
@@ -75,7 +75,7 @@ Wenn ein Branch geschützt ist, trifft Folgendes zu:
- Wenn erforderliche Pull-Request-Reviews auf dem Branch aktiviert sind, kannst Du Änderungen erst dann in den Branch zusammenführen, wenn alle Anforderungen der Richtlinie für Pull-Request-Reviews erfüllt sind. Weitere Informationen findest Du unter „[Einen Pull Request zusammenführen](/articles/merging-a-pull-request).“
- Wenn der erforderliche Review von einem Codeinhaber auf einem Branch aktiviert ist und der Code mit einem Inhaber durch einen Pull Request geändert wird, muss ein Codeinhaber den Pull Request genehmigen, bevor er zusammengeführt werden kann. Weitere Informationen findest Du unter „[Informationen zu Codeinhabern](/articles/about-code-owners).“
- Wenn die obligatorische Commit-Signatur auf einem Branch aktiviert ist, kannst Du keine Commits an den Branch übertragen, die nicht signiert und verifiziert sind. For more information, see "[About commit signature verification](/articles/about-commit-signature-verification)" and "[About required commit signing](/articles/about-required-commit-signing)."{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
-- Wenn du den Konflikt-Editor von {% data variables.product.prodname_dotcom %} benutzt, um Konflikte für eine Pull Request zu beheben, die Du aus einem geschützten Branch erstellt hast, wird Dir {% data variables.product.prodname_dotcom %} helfen, einen alternativen Branch für den Pull-Request zu erstellen, so dass Deine Auflösung der Konflikte zusammengeführt werden kann. Weitere Informationen findest Du unter „[Einen Mergekonflikt auf {% data variables.product.prodname_dotcom %} beheben](/github/collaborating-with-issues-and-pull-requests/resolving-a-merge-conflict-on-github).“{% endif %}
+- If you use {% data variables.product.prodname_dotcom %}'s conflict editor to fix conflicts for a pull request that you created from a protected branch, {% data variables.product.prodname_dotcom %} helps you to create an alternative branch for the pull request, so that your resolution of the conflicts can be merged. Weitere Informationen findest Du unter „[Einen Mergekonflikt auf {% data variables.product.prodname_dotcom %} beheben](/github/collaborating-with-issues-and-pull-requests/resolving-a-merge-conflict-on-github).“{% endif %}
### Weiterführende Informationen
diff --git a/translations/de-DE/content/github/collaborating-with-issues-and-pull-requests/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility.md b/translations/de-DE/content/github/collaborating-with-issues-and-pull-requests/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility.md
index 347a19a756fc..17821366dbbc 100644
--- a/translations/de-DE/content/github/collaborating-with-issues-and-pull-requests/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility.md
+++ b/translations/de-DE/content/github/collaborating-with-issues-and-pull-requests/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility.md
@@ -16,14 +16,20 @@ versions:
Wenn Du ein privates Repository löschst, werden alle zugehörigen privaten Forks ebenfalls gelöscht.
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
#### Öffentliches Repository löschen
Wenn Du ein öffentliches Repository löschst, wird einer der vorhandenen öffentlichen Forks als das neue übergeordnete Repository ausgewählt. Alle anderen Repositorys werden von diesem neuen übergeordneten Element abgezweigt, und nachfolgende Pull Requests werden an dieses neue übergeordnete Element gesendet.
+{% endif %}
+
#### Private Forks und Berechtigungen
{% data reusables.repositories.private_forks_inherit_permissions %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
#### Öffentliches Repository in ein privates Repository ändern
Wenn ein öffentliches Repository auf privat festgelegt wird, werden die zugehörigen öffentlichen Forks in ein neues Netzwerk abgespalten. Wie beim Löschen eines öffentlichen Repositorys wird einer der vorhandenen öffentliches Forks als das neue übergeordnete Repository ausgewählt, und alle anderen Repositorys werden von diesem neuen übergeordneten Element abgezweigt. Nachfolgende Pull Requests werden an dieses neue übergeordnete Element gesendet.
@@ -40,15 +46,36 @@ Wenn ein öffentliches Repository auf privat festgelegt und anschließend gelös
#### Privates Repository in ein öffentliches Repository ändern
-Wenn ein privates Repository auf öffentlich festgelegt wird, werden alle privaten Forks in eigenständige private Repositorys umgewandelt und werden zum übergeordneten Element ihres eigenen neuen Repository-Netzwerks. Private Forks werden niemals automatisch auf öffentlich festgelegt, da sie sensible Commits enthalten können, die nicht veröffentlicht werden sollten.
+Wenn ein privates Repository auf öffentlich festgelegt wird, werden alle privaten Forks in ein eigenständiges privates Repository umgewandelt und avancieren zum übergeordneten Element des eigenen neuen Repository-Netzwerks. Private Forks werden niemals automatisch auf öffentlich festgelegt, da sie sensible Commits enthalten können, die nicht veröffentlicht werden sollten.
##### Öffentliches Repository löschen
Wenn ein privates Repository auf öffentlich festgelegt und anschließend gelöscht wird, bleiben die zugehörigen privaten Forks in separaten Netzwerken als eigenständige private Repositorys erhalten.
+{% endif %}
+
+{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %}
+
+#### Changing the visibility of an internal repository
+
+{% note %}
+
+**Note:** {% data reusables.gated-features.internal-repos %}
+
+{% endnote %}
+
+If the policy for your enterprise permits forking, any fork of an internal repository will be private. If you change the visibility of an internal repository, any fork owned by an organization or user account will remain private.
+
+##### Deleting the internal repository
+
+If you change the visibility of an internal repository and then delete the repository, the forks will continue to exist in a separate network.
+
+{% endif %}
+
### Weiterführende Informationen
- „[Sichtbarkeit eines Repositorys festlegen](/articles/setting-repository-visibility)“
- „[Informationen zu Forks](/articles/about-forks)“
- „[Die Forking-Richtlinie für Dein Repository verwalten](/github/administering-a-repository/managing-the-forking-policy-for-your-repository)"
- „[Die Forking-Richtlinie für Deine Organisation verwalten](/github/setting-up-and-managing-organizations-and-teams/managing-the-forking-policy-for-your-organization)"
+- "{% if currentVersion == "free-pro-team@latest" %}[Enforcing repository management policies in your enterprise account](/github/setting-up-and-managing-your-enterprise/enforcing-repository-management-policies-in-your-enterprise-account#enforcing-a-policy-on-forking-private-or-internal-repositories){% else %}[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#enforcing-a-policy-on-forking-private-or-internal-repositories){% endif %}"
diff --git a/translations/de-DE/content/github/creating-cloning-and-archiving-repositories/about-readmes.md b/translations/de-DE/content/github/creating-cloning-and-archiving-repositories/about-readmes.md
index 2a00f5faac77..dfd2265ecfa7 100644
--- a/translations/de-DE/content/github/creating-cloning-and-archiving-repositories/about-readmes.md
+++ b/translations/de-DE/content/github/creating-cloning-and-archiving-repositories/about-readmes.md
@@ -11,7 +11,11 @@ versions:
github-ae: '*'
---
-A README file, along with {% if currentVersion == "free-pro-team@latest" %}a [repository license](/articles/licensing-a-repository), [contribution guidelines](/articles/setting-guidelines-for-repository-contributors), and a [code of conduct](/articles/adding-a-code-of-conduct-to-your-project){% else %}a [repository license](/articles/licensing-a-repository) and [contribution guidelines](/articles/setting-guidelines-for-repository-contributors){% endif %}, helps you communicate expectations for and manage contributions to your project.
+### Informationen zu README-Dateien
+
+You can add a README file to a repository to communicate important information about your project. A README, along with a repository license{% if currentVersion == "free-pro-team@latest" %}, contribution guidelines, and a code of conduct{% elsif enterpriseServerVersions contains currentVersion %} and contribution guidelines{% endif %}, communicates expectations for your project and helps you manage contributions.
+
+For more information about providing guidelines for your project, see {% if currentVersion == "free-pro-team@latest" %}"[Adding a code of conduct to your project](/github/building-a-strong-community/adding-a-code-of-conduct-to-your-project)" and {% endif %}"[Setting up your project for healthy contributions](/github/building-a-strong-community/setting-up-your-project-for-healthy-contributions)."
Die README-Datei ist oft das erste Element, das ein Benutzer beim Besuch Deines Repositorys sieht. README-Dateien enthalten in der Regel folgende Informationen:
- Was ist die Aufgabe des Projekts?
@@ -26,8 +30,12 @@ Wenn Sie Ihre README-Datei im Stammverzeichnis, im Ordner `docs` oder im verborg
{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21"%}
+
{% data reusables.profile.profile-readme %}
+{% endif %}
+

{% endif %}
diff --git a/translations/de-DE/content/github/creating-cloning-and-archiving-repositories/licensing-a-repository.md b/translations/de-DE/content/github/creating-cloning-and-archiving-repositories/licensing-a-repository.md
index a20368ac0aeb..4466fa063232 100644
--- a/translations/de-DE/content/github/creating-cloning-and-archiving-repositories/licensing-a-repository.md
+++ b/translations/de-DE/content/github/creating-cloning-and-archiving-repositories/licensing-a-repository.md
@@ -7,7 +7,6 @@ redirect_from:
versions:
free-pro-team: '*'
enterprise-server: '*'
- github-ae: '*'
---
### Die richtige Lizenz auswählen
@@ -18,7 +17,7 @@ Du bist nicht dazu verpflichtet, eine Lizenz auszuwählen. Bedenke jedoch, dass
{% note %}
-**Note:** If you publish your source code in a public repository on GitHub, {% if currentVersion == "free-pro-team@latest" %}according to the [Terms of Service](/articles/github-terms-of-service), {% endif %}other GitHub users have the right to view and fork your repository within the GitHub site. Wenn Du bereits ein öffentliches Repository erstellt hast und nicht mehr möchtest, dass andere Benutzer darauf zugreifen, kannst Du festlegen, dass Dein Repository privat ist. Wenn Du ein öffentliches Repository in ein privates umwandelst, bleiben vorhandene Forks oder lokale Kopien bestehen, die andere Benutzer erstellt haben. Weitere Informationen findest Du unter „[Ein öffentliches Repository in ein privates umwandeln](/articles/making-a-public-repository-private).“
+**Note:** If you publish your source code in a public repository on {% data variables.product.product_name %}, {% if currentVersion == "free-pro-team@latest" %}according to the [Terms of Service](/articles/github-terms-of-service), {% endif %}other users of {% data variables.product.product_location %} have the right to view and fork your repository. If you have already created a repository and no longer want users to have access to the repository, you can make the repository private. When you change the visibility of a repository to private, existing forks or local copies created by other users will still exist. Weitere Informationen findest Du unter „[Sichtbarkeit eines Repositorys festlegen](/github/administering-a-repository/setting-repository-visibility).“
{% endnote %}
diff --git a/translations/de-DE/content/github/developing-online-with-codespaces/using-codespaces-in-visual-studio.md b/translations/de-DE/content/github/developing-online-with-codespaces/using-codespaces-in-visual-studio.md
index 2f7d40e74ca1..4ce2419023a0 100644
--- a/translations/de-DE/content/github/developing-online-with-codespaces/using-codespaces-in-visual-studio.md
+++ b/translations/de-DE/content/github/developing-online-with-codespaces/using-codespaces-in-visual-studio.md
@@ -46,8 +46,12 @@ Connecting to {% data variables.product.prodname_github_codespaces %} with the
### Configuring a codespace for {% data variables.product.prodname_vs %}
-The default codespace environment created by {% data variables.product.prodname_vs %} includes popular frameworks and tools such as .NET Core, Microsoft SQL Server, Python, and the Windows SDK. {% data variables.product.prodname_github_codespaces %} created with {% data variables.product.prodname_vs %} can be customized through a subset of `devcontainers.json` properties and a new tool called devinit, included with {% data variables.product.prodname_vs %}.
+A codespace, created with {% data variables.product.prodname_vs %}, can be customized through a new tool called devinit, a command line tool included with {% data variables.product.prodname_vs %}.
#### devinit
-The [devinit](https://docs.microsoft.com/visualstudio/devinit/getting-started-with-devinit) command-line tool lets you install additional frameworks and tools into your Windows development codespaces, as well as run PowerShell scripts or modify environment variables. devinit supports a configuration file called [devinit.json](https://docs.microsoft.com/visualstudio/devinit/devinit-json), which can be added to your project for creating customized and repeatable development environments. For more information about Windows codespace configuration and devinit, see [Customize a codespace](https://docs.microsoft.com/visualstudio/ide/codespaces/customize-codespaces) in the {% data variables.product.prodname_vs %} documentation.
+[devinit](https://docs.microsoft.com/visualstudio/devinit/getting-started-with-devinit) lets you install additional frameworks and tools into your Windows development codespaces, modify environment variables, and more.
+
+devinit supports a configuration file called [devinit.json](https://docs.microsoft.com/visualstudio/devinit/devinit-json). You can add this file to your project if you want to create a customized and repeatable development environment. When you use devinit with a [devcontainer.json](https://docs.microsoft.com/visualstudio/ide/codespaces/customize-codespaces#running-devinit-when-creating-a-codespace) file, your codespaces will be automatically configured on creation.
+
+For more information about Windows codespace configuration and devinit, see [Customize a codespace](https://docs.microsoft.com/visualstudio/ide/codespaces/customize-codespaces) in the {% data variables.product.prodname_vs %} documentation. For more information about devinit, see [Getting started with devinit](https://docs.microsoft.com/visualstudio/devinit/getting-started-with-devinit).
diff --git a/translations/de-DE/content/github/getting-started-with-github/create-a-repo.md b/translations/de-DE/content/github/getting-started-with-github/create-a-repo.md
index 6b34e0a51c33..e9997b601dd1 100644
--- a/translations/de-DE/content/github/getting-started-with-github/create-a-repo.md
+++ b/translations/de-DE/content/github/getting-started-with-github/create-a-repo.md
@@ -10,14 +10,26 @@ versions:
github-ae: '*'
---
+{% if currentVersion == "free-pro-team@latest" %}
+
Sie können die unterschiedlichsten Projekte in {% data variables.product.product_name %}-Repositorys speichern, darunter auch Open-Source-Projekte. Mit [Open-Source-Projekten](http://opensource.org/about) kannst Du Code leichter für andere zugänglich machen, um eine bessere, zuverlässigere Software zu entwickeln.
+{% elsif enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
+
+You can store a variety of projects in {% data variables.product.product_name %} repositories, including innersource projects. With innersource, you can share code to make better, more reliable software. Weitere Informationen zu InnerSource finden Sie im Whitepaper „[An introduction to innersource](https://resources.github.com/whitepapers/introduction-to-innersource/)“ (Einführung in InnerSource) von {% data variables.product.company_short %}.
+
+{% endif %}
+
+{% if currentVersion == "free-pro-team@latest" %}
+
{% note %}
**Hinweis:** Du kannst öffentliche Repositorys für ein Open-Source-Projekt erstellen. Wenn Du ein öffentliches Repository erstellst, musst du unbedingt eine [Lizenzdatei](http://choosealicense.com/) hinzufügen, die bestimmt, wie Dein Projekt für andere Personen freigegeben wird. {% data reusables.open-source.open-source-guide-repositories %} {% data reusables.open-source.open-source-learning-lab %}
{% endnote %}
+{% endif %}
+
{% data reusables.repositories.create_new %}
2. Gib einen kurzen, leicht merkbaren Namen für Dein Repository ein. Beispiel: „hello world“. 
3. Optional kannst Du auch eine Beschreibung des Repositorys hinzufügen. For example, "My first repository on
diff --git a/translations/de-DE/content/github/getting-started-with-github/fork-a-repo.md b/translations/de-DE/content/github/getting-started-with-github/fork-a-repo.md
index f3551a6dd339..c24b44827fda 100644
--- a/translations/de-DE/content/github/getting-started-with-github/fork-a-repo.md
+++ b/translations/de-DE/content/github/getting-started-with-github/fork-a-repo.md
@@ -25,10 +25,16 @@ Du kannst Forks beispielsweise benutzen, um Änderungen im Zusammenhang mit der
Open-Source-Software basiert auf der Idee, dass wir durch gemeinsamen Code bessere, zuverlässigere Software erstellen können. Weitere Informationen findest Du in „[Über die Open-Source-Initiative](http://opensource.org/about)“ auf der Open-Source-Initiative-Website.
+For more information about applying open source principles to your organization's development work on {% data variables.product.product_location %}, see {% data variables.product.prodname_dotcom %}'s whitepaper "[An introduction to innersource](https://resources.github.com/whitepapers/introduction-to-innersource/)."
+
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
Wenn Du ein öffentliches Repository von einem Fork eines Projekts eines anderen Benutzers erstellst, musst Du unbedingt eine Lizenzdatei hinzufügen, die bestimmt, wie Dein Projekt für andere Personen freigegeben wird. Weitere Informationen findest Du unter „[Wähle eine Open-Source-Lizenz](http://choosealicense.com/)“ auf der choosealicense-Website.
{% data reusables.open-source.open-source-guide-repositories %} {% data reusables.open-source.open-source-learning-lab %}
+{% endif %}
+
{% note %}
**Hinweis**: {% data reusables.repositories.desktop-fork %}
diff --git a/translations/de-DE/content/github/getting-started-with-github/setting-up-a-trial-of-github-enterprise-server.md b/translations/de-DE/content/github/getting-started-with-github/setting-up-a-trial-of-github-enterprise-server.md
index 894f6f6dd288..f3c95525bad7 100644
--- a/translations/de-DE/content/github/getting-started-with-github/setting-up-a-trial-of-github-enterprise-server.md
+++ b/translations/de-DE/content/github/getting-started-with-github/setting-up-a-trial-of-github-enterprise-server.md
@@ -36,7 +36,7 @@ Um Deine Testversion optimal zu nutzen, folge diesen Schritten:
- Webcast „[Kurzanleitung zu {% data variables.product.prodname_dotcom %}](https://resources.github.com/webcasts/Quick-start-guide-to-GitHub/)“
- „[Verstehen des {% data variables.product.prodname_dotcom %}-Ablaufs](https://guides.github.com/introduction/flow/)“ unter „{% data variables.product.prodname_dotcom %}-Anleitungen“
- „[Hello World](https://guides.github.com/activities/hello-world/)“ unter „{% data variables.product.prodname_dotcom %}-Anleitungen“
-3. To configure your instance to meet your organization's needs, see "[Configuring your enterprise](/admin/configuration/configuring-your-enterprise)."
+3. To configure your instance to meet your organization's needs, see "[Configuring your enterprise](/enterprise/admin/configuration/configuring-your-enterprise)."
4. Integrieren Sie {% data variables.product.prodname_ghe_server %} mit Ihrem Identity Provider. Details finden Sie unter „[SAML verwenden](/enterprise/admin/user-management/using-saml)“ und „[LDAP verwenden](/enterprise/admin/authentication/using-ldap)“.
5. Lade beliebig viele Personen zum Test ein.
- Füge die Benutzer zu Deiner {% data variables.product.prodname_ghe_server %}-Instanz hinzu, entweder mit der integrierten Authentifizierung oder Deinem konfigurierten Identitätsanbieter. Weitere Informationen findest Du unter „[Integrierte Authentifizierung verwenden](/enterprise/admin/user-management/using-built-in-authentication).“
diff --git a/translations/de-DE/content/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies.md b/translations/de-DE/content/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies.md
index 28e69946ffbc..5ed4ecd34c90 100644
--- a/translations/de-DE/content/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies.md
+++ b/translations/de-DE/content/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies.md
@@ -75,7 +75,7 @@ You can see all of the alerts that affect a particular project{% if currentVersi
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
By default, we notify people with admin permissions in the affected repositories about new
-{% data variables.product.prodname_dependabot_alerts %}.{% endif %} {% if currentVersion == "free-pro-team@latest" %}{% data variables.product.product_name %} never publicly discloses identified vulnerabilities for any repository. You can also make {% data variables.product.prodname_dependabot_alerts %} visible to additional people or teams working repositories that you own or have admin permissions for. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-dependabot-alerts)."
+{% data variables.product.prodname_dependabot_alerts %}.{% endif %} {% if currentVersion == "free-pro-team@latest" %}{% data variables.product.product_name %} never publicly discloses identified vulnerabilities for any repository. You can also make {% data variables.product.prodname_dependabot_alerts %} visible to additional people or teams working repositories that you own or have admin permissions for. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-security-alerts)."
{% endif %}
{% if enterpriseServerVersions contains currentVersion and currentVersion ver_lt "enterprise-server@2.22" %}
diff --git a/translations/de-DE/content/github/managing-subscriptions-and-notifications-on-github/about-notifications.md b/translations/de-DE/content/github/managing-subscriptions-and-notifications-on-github/about-notifications.md
index 2ae86832280c..e5902fcbd6c1 100644
--- a/translations/de-DE/content/github/managing-subscriptions-and-notifications-on-github/about-notifications.md
+++ b/translations/de-DE/content/github/managing-subscriptions-and-notifications-on-github/about-notifications.md
@@ -21,7 +21,12 @@ Du kannst Benachrichtigungen abonnieren für:
- Eine Unterhaltung in einem spezifischen Issue, Pull Request oder Gist.
- Alle Aktivitäten in einem Repository oder in einer Team-Diskussion.
- CI-Aktivität wie beispielsweise der Status von Workflows in Repositorys, die mit {% data variables.product.prodname_actions %} aufgesetzt wurden.
+{% if currentVersion == "free-pro-team@latest" %}
+- Issues, pulls requests, releases and discussions (if enabled) in a repository.
+{% endif %}
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}
- Releases in einem Repository.
+{% endif %}
Du kannst auch automatisch alle Repositorys überwachen, auf die Du Push-Zugriff hast, mit Ausnahme von Forks. Du kannst jedes andere Repository, auf das Du Zugriff hast, manuell verfolgen durch klicken auf **Watch** (Beobachten).
diff --git a/translations/de-DE/content/github/managing-subscriptions-and-notifications-on-github/configuring-notifications.md b/translations/de-DE/content/github/managing-subscriptions-and-notifications-on-github/configuring-notifications.md
index 1bfba80f063b..fd0b772b8f06 100644
--- a/translations/de-DE/content/github/managing-subscriptions-and-notifications-on-github/configuring-notifications.md
+++ b/translations/de-DE/content/github/managing-subscriptions-and-notifications-on-github/configuring-notifications.md
@@ -21,16 +21,17 @@ versions:
### Zustellungsoptionen für Benachrichtigungen
-Du hast drei grundlegende Optionen für die Zustellung von Benachrichtigungen:
- - the notifications inbox on {% data variables.product.product_name %}{% if currentVersion == "free-pro-team@latest" %}
- - the notifications inbox on {% data variables.product.prodname_mobile %}, which syncs with the inbox on {% data variables.product.product_name %}{% endif %}
- - an email client that uses a verified email address, which can also sync with the notifications inbox on {% data variables.product.product_name %}{% if currentVersion == "free-pro-team@latest" %} and {% data variables.product.prodname_mobile %}{% endif %}
+You can receive notifications for activity on {% data variables.product.product_name %} in the following locations.
+
+ - The notifications inbox in the {% data variables.product.product_name %} web interface{% if currentVersion == "free-pro-team@latest" %}
+ - The notifications inbox on {% data variables.product.prodname_mobile %}, which syncs with the inbox on {% data variables.product.product_name %}{% endif %}
+ - An email client that uses a verified email address, which can also sync with the notifications inbox on {% data variables.product.product_name %}{% if currentVersion == "free-pro-team@latest" %} and {% data variables.product.prodname_mobile %}{% endif %}
{% if currentVersion == "free-pro-team@latest" %}
{% data reusables.notifications-v2.notifications-inbox-required-setting %} Weitere Informationen findest Du auf „[Deine Benachrichtigungseinstellungen wählen](#choosing-your-notification-settings)."
{% endif %}
-{% data reusables.notifications-v2.tip-for-syncing-email-and-your-inbox-on-github %}
+{% data reusables.notifications.shared_state %}
#### Benefits of the notifications inbox
@@ -59,8 +60,21 @@ E-Mail-Benachrichtigungen ermöglichen auch Flexibilität bei der Art von Benach
### Über die Teilnahme und das Beobachten von Benachrichtigungen
-Wenn Du ein Repository beobachtest, abonnierst Du Aktualisierungen für Aktivitäten in diesem Repository. Ebenfalls, wenn Du die Diskussionen eines bestimmten Teams verfolgst, abonnierst Du alle Aktualisierungen der Unterhaltung auf der Seite dieses Teams. Um Repositorys zu sehen, die Du beobachtest, siehe [https://github.com/watching](https://github.com/watching). Weitere Informationen findest Du unter „[Abonnements und Benachrichtigungen auf GitHub verwalten](/github/managing-subscriptions-and-notifications-on-github/managing-subscriptions-for-activity-on-github)."
+Wenn Du ein Repository beobachtest, abonnierst Du Aktualisierungen für Aktivitäten in diesem Repository. Ebenfalls, wenn Du die Diskussionen eines bestimmten Teams verfolgst, abonnierst Du alle Aktualisierungen der Unterhaltung auf der Seite dieses Teams. Weitere Informationen finden Sie unter „[Informationen zu Teamdiskussionen](/github/building-a-strong-community/about-team-discussions)“.
+
+To see repositories that you're watching, go to your [watching page](https://github.com/watching). Weitere Informationen findest Du unter „[Abonnements und Benachrichtigungen auf GitHub verwalten](/github/managing-subscriptions-and-notifications-on-github/managing-subscriptions-for-activity-on-github)."
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}
+#### Benachrichtigungen konfigurieren
+{% endif %}
+You can configure notifications for a repository on the repository page, or on your watching page.
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %} You can choose to only receive notifications for releases in a repository, or ignore all notifications for a repository.{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+
+#### About custom notifications
+{% data reusables.notifications-v2.custom-notifications-beta %}
+You can customize notifications for a repository, for example, you can choose to only be notified when updates to one or more types of events (issues, pull request, releases, discussions) happen within a repository, or ignore all notifications for a repository.
+{% endif %} For more information, see "[Viewing your subscriptions](/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions#configuring-your-watch-settings-for-an-individual-repository)."
+#### Participating in conversations
Jedes Mal, wenn Du in einer Unterhaltung kommentierst oder wenn jemand Deinen Benutzernamen @erwähnt, bist Du _Teilnehmer_ in einer Unterhaltung. Standardmäßig abonnierst Du automatisch eine Unterhaltung, wenn Du daran teilnimmst. Du kannst Dich manuell von einer Unterhaltung abmelden, an der Du teilgenommen hast, indem Du auf dem Issue oder Pull Request auf **Unsubscribe** (Abmelden) klickst oder durch die Option **Unsubscribe** (Abmelden) im Posteingang für Benachrichtigungen.
For conversations you're watching or participating in, you can choose whether you want to receive notifications by email or through the notifications inbox on {% data variables.product.product_name %}{% if currentVersion == "free-pro-team@latest" %} and {% data variables.product.prodname_mobile %}{% endif %}.
@@ -95,7 +109,9 @@ Wähle eine Standard-E-Mail-Adresse, an die Du Aktualisierungen für Unterhaltun
- Pull-Request-Pushes.
- Deine eigenen Aktualisierungen, wie wenn Du beispielsweise einen Issue oder Pull Request öffnest, kommentierst oder schließt.
-Abhängig von der Organisation, die das Repository besitzt, kannst Du auch Benachrichtigungen für bestimmte Repositories an verschiedene E-Mail-Adressen senden. Beispielsweise kannst Du Benachrichtigungen für ein bestimmtes öffentliches Repository an eine verifizierte persönliche E-Mail-Adresse senden. Deine Organisation verlangt möglicherweise, dass E-Mail-Adressen für bestimmte Domänen verifiziert sein müssen. Weitere Informationen findest Du auf „[Auswählen, wohin die E-Mail-Benachrichtigungen Deiner Organisation gesendet werden](/github/managing-subscriptions-and-notifications-on-github/configuring-notifications#choosing-where-your-organizations-email-notifications-are-sent)."
+Depending on the organization that owns the repository, you can also send notifications to different email addresses. Deine Organisation verlangt möglicherweise, dass E-Mail-Adressen für bestimmte Domänen verifiziert sein müssen. For more information, see "[Choosing where your organization’s email notifications are sent](/github/managing-subscriptions-and-notifications-on-github/configuring-notifications#choosing-where-your-organizations-email-notifications-are-sent)."
+
+You can also send notifications for a specific repository to an email address. Weitere Informationen findest Du unter "[Informationen zu E-Mail-Benachrichtigungen für Pushes in Dein Repository](/github/administering-a-repository/about-email-notifications-for-pushes-to-your-repository)."
{% data reusables.notifications-v2.email-notification-caveats %}
diff --git a/translations/de-DE/content/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions.md b/translations/de-DE/content/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions.md
index 9b4938a566f2..4f03a25e7492 100644
--- a/translations/de-DE/content/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions.md
+++ b/translations/de-DE/content/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions.md
@@ -55,6 +55,13 @@ Wenn Du ein Repository nicht mehr beobachtest, meldest Du Dich von zukünftigen
{% data reusables.notifications.access_notifications %}
1. Verwende in der linken Seitenleiste, unterhalb der Liste der Repositorys, das Dropdownmenü „Manage Notifications" (Benachrichtigungen verwalten) und klicke auf **Watched repositories** (beobachtete Repositorys). 
2. Nimm auf der Seite der beobachteten Repositorys eine Bewertung dieser Repositorys vor und wähle dann aus:
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}
- ein Repository nicht mehr beobachten
- nur Releases für ein Repository beobachten
- ignoriere alle Benachrichtigungen für ein Repository
+{% endif %}
+{% if currentVersion == "free-pro-team@latest" %}
+ - ein Repository nicht mehr beobachten
+ - ignoriere alle Benachrichtigungen für ein Repository
+ - customize the types of event you receive notifications for (issues, pull requests, releases or discussions, if enabled)
+{% endif %}
\ No newline at end of file
diff --git a/translations/de-DE/content/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions.md b/translations/de-DE/content/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions.md
index fcc3fd8391e1..e5087e87aa2f 100644
--- a/translations/de-DE/content/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions.md
+++ b/translations/de-DE/content/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions.md
@@ -32,7 +32,16 @@ Wenn Dein Posteingang zu viele Benachrichtigungen zum Verwalten hat, überlege D
Weitere Informationen findest Du unter „[Benachrichtigungen konfigurieren](/github/managing-subscriptions-and-notifications-on-github/configuring-notifications#automatic-watching)."
-Eine Übersicht Deiner Repository-Abonnements findest Du unter „[Repositorys überprüfen, die Du beobachtest](#reviewing-repositories-that-youre-watching). Viele Personen vergessen Repositorys, die sie in der Vergangenheit beobachtet haben. Über die Seite „Watched repositories" (beobachtete Repositorys) kannst Du die Beobachtung von Repositorys schnell deaktivieren. Weitere Informationen über Deine Abmeldeoptionen findest Du unter „[Abonnements verwalten](/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions)."
+Eine Übersicht Deiner Repository-Abonnements findest Du unter „[Repositorys überprüfen, die Du beobachtest](#reviewing-repositories-that-youre-watching).
+{% if currentVersion == "free-pro-team@latest" %}
+{% tip %}
+
+**Tip:** You can select the types of event to be notified of by using the **Custom** option of the **Watch/Unwatch** dropdown list in your [watching page](https://github.com/watching) or on any repository page on {% data variables.product.prodname_dotcom_the_website %}. For more information, see "[Configuring your watch settings for an individual repository](#configuring-your-watch-settings-for-an-individual-repository)" below.
+
+{% endtip %}
+{% endif %}
+
+Viele Personen vergessen Repositorys, die sie in der Vergangenheit beobachtet haben. Über die Seite „Watched repositories" (beobachtete Repositorys) kannst Du die Beobachtung von Repositorys schnell deaktivieren. For more information on ways to unsubscribe, see "[Unwatch recommendations](https://github.blog/changelog/2020-11-10-unwatch-recommendations/)" on {% data variables.product.prodname_blog %} and "[Managing your subscriptions](/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions)." You can also create a triage worflow to help with the notifications you receive. For guidance on triage workflows, see "[Customizing a workflow for triaging your notifications](/github/managing-subscriptions-and-notifications-on-github/customizing-a-workflow-for-triaging-your-notifications)."
### Alle Deine Abonnements überprüfen
@@ -55,20 +64,38 @@ Eine Übersicht Deiner Repository-Abonnements findest Du unter „[Repositorys
### Repositorys überprüfen, die Du beobachtest
1. Verwende in der linken Seitenleiste, unterhalb der Liste der Repositorys, das Dropdownmenü „Manage Notifications" (Benachrichtigungen verwalten) und klicke auf **Watched repositories** (beobachtete Repositorys). 
-
-3. Evaluiere die von Dir beobachteten Repositorys und entscheide, ob deren Aktualisierungen für Dich immer noch relevant und hilfreich sind. Wenn Du ein Repository beobachtest, wirst Du über alle Unterhaltungen für dieses Repository benachrichtigt.
-
+2. Evaluiere die von Dir beobachteten Repositorys und entscheide, ob deren Aktualisierungen für Dich immer noch relevant und hilfreich sind. Wenn Du ein Repository beobachtest, wirst Du über alle Unterhaltungen für dieses Repository benachrichtigt.
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}

+{% endif %}
+{% if currentVersion == "free-pro-team@latest" %}
+ 
+{% endif %}
{% tip %}
- **Tipp:** Anstatt ein Repository zu beobachten, erwäge allenfalls nur Releases für ein Repository ansehen oder ein Repository gar nicht mehr zu beobachten. Wenn Du ein Repository nicht mehr beobachtest, kannst du immer noch benachrichtigt werden, wenn Du @erwähnt wirst oder in einem Thread teilnimmst. Wenn Du nur Releases für ein Repository beobachtest, wirst Du nur benachrichtigt, wenn es einen neuen Release gibt, wenn Du an einem Thread teilnimmst oder wenn Du oder ein Team, dem Du angehörst, @erwähnt wirst.
+ **Tip:** Instead of watching a repository, consider only receiving notifications {% if currentVersion == "free-pro-team@latest" %}when there are updates to issues, pull requests, releases or discussions (if enabled for the repository), or any combination of these options,{% else %}for releases in a repository,{% endif %} or completely unwatching a repository.
+
+ Wenn Du ein Repository nicht mehr beobachtest, kannst du immer noch benachrichtigt werden, wenn Du @erwähnt wirst oder in einem Thread teilnimmst. When you configure to receive notifications for certain event types, you're only notified when there are updates to these event types in the repository, you're participating in a thread, or you or a team you're on is @mentioned.
{% endtip %}
### Konfiguration der Beobachtungseinstellungen für ein einzelnes Repository
-Du kannst wählen, ob Du ein einzelnes Repository ansehen möchtest oder nicht mehr. Du kannst auch wählen, dass Du nur bei neuen Releases benachrichtigt wirst oder Du kannst ein einzelnes Repository komplett ignorieren.
+Du kannst wählen, ob Du ein einzelnes Repository ansehen möchtest oder nicht mehr. You can also choose to only be notified of {% if currentVersion == "free-pro-team@latest" %}certain event types such as issues, pull requests, discussions (if enabled for the repository) and {% endif %}new releases, or completely ignore an individual repository.
{% data reusables.repositories.navigate-to-repo %}
-2. Klicke in der oberen rechten Ecke auf das Dropdownmenü „Watch" (Beobachten), um eine der Beobachtungsoptionen zu wählen. 
+2. Klicke in der oberen rechten Ecke auf das Dropdownmenü „Watch" (Beobachten), um eine der Beobachtungsoptionen zu wählen.
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}
+ 
+{% endif %}
+{% if currentVersion == "free-pro-team@latest" %}
+ 
+{% data reusables.notifications-v2.custom-notifications-beta %}
+The **Custom** option allows you to further customize notifications so that you're only notified when specific events happen in the repository, in addition to participating and @mentions.
+
+ 
+
+If you select "Issues", you will be notified about, and subscribed to, updates on every issue (including those that existed prior to you selecting this option) in the repository. If you're @mentioned in a pull request in this repository, you'll receive notifications for that too, and you'll be subscribed to updates on that specific pull request, in addition to being notified about issues.
+
+{% endif %}
diff --git a/translations/de-DE/content/github/managing-your-work-on-github/creating-an-issue.md b/translations/de-DE/content/github/managing-your-work-on-github/creating-an-issue.md
index d9d6b00e33c5..c8f5ad83d2d9 100644
--- a/translations/de-DE/content/github/managing-your-work-on-github/creating-an-issue.md
+++ b/translations/de-DE/content/github/managing-your-work-on-github/creating-an-issue.md
@@ -1,6 +1,7 @@
---
title: Einen Issue erstellen
intro: 'Mit Issues kannst Du Fehler, Verbesserungen oder andere Anforderungen nachverfolgen.'
+permissions: 'People with read permissions can create an issue in a repository where issues are enabled.'
redirect_from:
- /articles/creating-an-issue
versions:
@@ -9,8 +10,6 @@ versions:
github-ae: '*'
---
-{% data reusables.repositories.create-issue-in-public-repository %}
-
Du kannst einen neuen Issue basierend auf dem Code eines vorhandenen Pull Requests erstellen. Weitere Informationen findest Du unter „[Einen Issue im Code öffnen](/github/managing-your-work-on-github/opening-an-issue-from-code).“
Du kannst einen neuen Issue direkt aus einem Kommentar in einem Issue- oder Pull-Request-Review öffnen. Weitere Informationen findest Du unter „[Öffnen eines Issue aus einem Kommentar](/github/managing-your-work-on-github/opening-an-issue-from-a-comment)."
diff --git a/translations/de-DE/content/github/managing-your-work-on-github/opening-an-issue-from-a-comment.md b/translations/de-DE/content/github/managing-your-work-on-github/opening-an-issue-from-a-comment.md
index 733aaa8bb5bc..00394cbafaba 100644
--- a/translations/de-DE/content/github/managing-your-work-on-github/opening-an-issue-from-a-comment.md
+++ b/translations/de-DE/content/github/managing-your-work-on-github/opening-an-issue-from-a-comment.md
@@ -1,6 +1,7 @@
---
title: Einen Issue aus einem Kommentar öffnen
intro: Du kannst einen neuen Issue aus einem spezifischen Kommentar in einem Issue oder Pull Request öffnen.
+permissions: 'People with read permissions can create an issue in a repository where issues are enabled.'
versions:
free-pro-team: '*'
enterprise-server: '*'
@@ -9,6 +10,8 @@ versions:
Wenn Du einen Issue aus einem Kommentar öffnest, wird der Issue ein Ausschnitt enthalten, der zeigt, wo der Kommentar ursprünglich eingestellt wurde.
+{% data reusables.repositories.administrators-can-disable-issues %}
+
1. Navigiere zum Kommentar, aus dem Du einen Issue öffnen möchtest.
2. Klicke in diesem Kommentar auf {% octicon "kebab-horizontal" aria-label="The horizontal kebab icon" %}. 
diff --git a/translations/de-DE/content/github/managing-your-work-on-github/opening-an-issue-from-code.md b/translations/de-DE/content/github/managing-your-work-on-github/opening-an-issue-from-code.md
index da000139e480..10e243e39f03 100644
--- a/translations/de-DE/content/github/managing-your-work-on-github/opening-an-issue-from-code.md
+++ b/translations/de-DE/content/github/managing-your-work-on-github/opening-an-issue-from-code.md
@@ -1,6 +1,7 @@
---
title: Einen Issue im Code öffnen
intro: Du kannst neue Issues von einer bestimmten Codezeile oder über mehreren Codezeilen in einer Datei oder einem Pull Request öffnen.
+permissions: 'People with read permissions can create an issue in a repository where issues are enabled.'
redirect_from:
- /articles/opening-an-issue-from-code
versions:
@@ -13,7 +14,7 @@ Wenn Du einen Issue im Code öffnest, enthält der Issue einen Ausschnitt mit de

-{% data reusables.repositories.create-issue-in-public-repository %}
+{% data reusables.repositories.administrators-can-disable-issues %}
{% data reusables.repositories.navigate-to-repo %}
2. Suche den Code, auf den Du in einem Issue verweisen möchtest:
diff --git a/translations/de-DE/content/github/managing-your-work-on-github/transferring-an-issue-to-another-repository.md b/translations/de-DE/content/github/managing-your-work-on-github/transferring-an-issue-to-another-repository.md
index 50b61fdffdc0..32fe48320e8c 100644
--- a/translations/de-DE/content/github/managing-your-work-on-github/transferring-an-issue-to-another-repository.md
+++ b/translations/de-DE/content/github/managing-your-work-on-github/transferring-an-issue-to-another-repository.md
@@ -11,7 +11,7 @@ versions:
Für die Übertragung eines offenen Issues in ein anderes Repository benötigst Du Schreibberechtigung sowohl für das Repository, aus dem der Issue stammt, wie auch für das Repository, in das der Issue übertragen wird. Weitere Informationen finden Sie unter„[Berechtigungsebenen für die Repositorys einer Organisation](/articles/repository-permission-levels-for-an-organization)“.
-Du kannst Issues nur zwischen Repositorys übertragen, die demselben Benutzer- oder Organisationskonto angehören. Du kannst einen Issue nicht aus einem privaten Repository in ein öffentliches Repository übertragen.
+You can only transfer issues between repositories owned by the same user or organization account.{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}You can't transfer an issue from a private repository to a public repository.{% endif %}
Wenn Du einen Issue überträgst, bleiben seine Kommentare und Bearbeiter erhalten. The issue's labels and milestones are not retained. This issue will stay on any user-owned or organization-wide project boards and be removed from any repository project boards. Weitere Informationen findest Du unter „[Informationen zu Projektboards](/articles/about-project-boards).“
diff --git a/translations/de-DE/content/github/searching-for-information-on-github/about-searching-on-github.md b/translations/de-DE/content/github/searching-for-information-on-github/about-searching-on-github.md
index 6efd7775f46e..1d31fb0a4df5 100644
--- a/translations/de-DE/content/github/searching-for-information-on-github/about-searching-on-github.md
+++ b/translations/de-DE/content/github/searching-for-information-on-github/about-searching-on-github.md
@@ -14,7 +14,7 @@ versions:
github-ae: '*'
---
-Du kannst global über {% data variables.product.product_name %} hinweg suchen oder Deine Suche auf ein bestimmtes Repositorys oder eine bestimmte Organisation beschränken.
+{% data reusables.search.you-can-search-globally %}
- Um global über {% data variables.product.product_name %} hinweg zu suchen, gib Deine Suchanfrage in das Suchfeld oben auf jeder Seite ein, und wähle im im Dropdownmenü der Suche „All {% data variables.product.prodname_dotcom %}“ (Ganzes Produkt).
- Um in einem bestimmten Repository oder einer bestimmten Organisation zu suchen, navigiere zur Repository- oder Organisationsseite, gib Deine Suchanfrage in das Suchfeld oben auf der Seite ein, und drücke die **Eingabetaste**.
@@ -23,20 +23,21 @@ Du kannst global über {% data variables.product.product_name %} hinweg suchen o
**Hinweise:**
-- {% data reusables.search.required_login %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- {% data reusables.search.required_login %}{% endif %}
- {% data variables.product.prodname_pages %}-Websites können auf {% data variables.product.product_name %} nicht durchsucht werden. Du kannst aber den Quellinhalt mithilfe der Codesuche durchsuchen, wenn er im Standardbranch eines Repositorys vorhanden ist. Weitere Informationen findest Du unter „[Code durchsuchen](/articles/searching-code)“. Weitere Informationen über {% data variables.product.prodname_pages %} findest Du unter „[Was ist GitHub Pages?](/articles/what-is-github-pages/)“
- Currently our search doesn't support exact matching.
- Whenever you are searching in code files, only the first two results in each file will be returned.
{% endnote %}
-Nach einer Suche auf {% data variables.product.product_name %} kannst Du die Ergebnisse sortieren oder durch Anklicken einer der Sprachen in der Seitenleiste weiter eingrenzen. Weitere Informationen findest Du unter „[Suchergebnisse sortieren](/articles/sorting-search-results).“
+Nach einer Suche auf {% data variables.product.product_name %} können Sie die Ergebnisse sortieren oder durch Anklicken einer der Sprachen in der Seitenleiste weiter eingrenzen. Weitere Informationen finden Sie unter „[Suchergebnisse sortieren](/articles/sorting-search-results)“.
-Bei der {% data variables.product.product_name %}-Suche wird ein ElasticSearch-Cluster verwendet, um Projekte jedes Mal zu indizieren, wenn eine Änderung an {% data variables.product.product_name %} übertragen wird. Issues und Pull Requests werden beim Anlegen oder Ändern indiziert.
+Bei der {% data variables.product.product_name %}-Suche wird ein ElasticSearch-Cluster verwendet, um Projekte jedes Mal zu indizieren, wenn eine Änderung an {% data variables.product.product_name %} gepusht wird. Issues und Pull Requests werden beim Anlegen oder Ändern indiziert.
### Arten von Suchen auf {% data variables.product.prodname_dotcom %}
-Du kannst die folgenden Arten von Informationen in allen öffentlichen {% data variables.product.product_name %}-Repositorys und in allen privaten {% data variables.product.product_name %}-Repositorys durchsuchen, auf die Du Zugriff hast:
+You can search for the following information across all repositories you can access on {% data variables.product.product_location %}.
- [Repositorys](/articles/searching-for-repositories)
- [Themen](/articles/searching-topics)
@@ -49,20 +50,20 @@ Du kannst die folgenden Arten von Informationen in allen öffentlichen {% data v
### Über eine visuelle Oberfläche suchen
-Alternativ kannst Du {% data variables.product.product_name %} mit der {% data variables.search.search_page_url %} oder {% data variables.search.advanced_url %} durchsuchen.
+Alternativ können Sie {% data variables.product.product_name %} mit der {% data variables.search.search_page_url %} oder {% data variables.search.advanced_url %} durchsuchen.
-Die {% data variables.search.advanced_url %} bietet eine visuelle Oberfläche zum Erstellen von Suchanfragen. Du kannst Deine Suchanfragen nach einer Vielzahl von Faktoren filtern, beispielsweise nach der Anzahl der Sterne oder der Anzahl der Forks eines Repositorys. Während Du die erweiterten Suchfelder ausfüllst, wird Deine Anfrage automatisch in der oberen Suchleiste erstellt.
+Die {% data variables.search.advanced_url %} bietet eine visuelle Oberfläche zum Erstellen von Suchanfragen. Sie können Ihre Suchanfragen nach einer Vielzahl von Faktoren filtern, beispielsweise nach der Anzahl der Sterne oder der Anzahl der Forks eines Repositorys. Wenn Sie die erweiterten Suchfelder ausfüllen, wird Ihre Anfrage automatisch in der oberen Suchleiste erstellt.

{% if currentVersion != "github-ae@latest" %}
### {% data variables.product.prodname_enterprise %} und {% data variables.product.prodname_dotcom_the_website %} gleichzeitig durchsuchen
-Wenn Sie {% data variables.product.prodname_enterprise %} verwenden und Sie Mitglied einer {% data variables.product.prodname_dotcom_the_website %}-Organisation sind, die {% data variables.product.prodname_ghe_cloud %} verwendet, kann Ihr {% data variables.product.prodname_enterprise %}-Websiteadministrator {% data variables.product.prodname_github_connect %} aktivieren, damit Sie beide Umgebungen gleichzeitig durchsuchen können. Weitere Informationen finden Sie unter „[{% data variables.product.prodname_unified_search %} zwischen {% data variables.product.prodname_enterprise %} und {% data variables.product.prodname_dotcom_the_website %} aktivieren](/enterprise/admin/guides/developer-workflow/enabling-unified-search-between-github-enterprise-server-and-github-com)“.
+Wenn Du {% data variables.product.prodname_enterprise %} verwendest und Mitglied einer {% data variables.product.prodname_dotcom_the_website %}-Organisation bist, die {% data variables.product.prodname_ghe_cloud %} verwendet, kann Dein {% data variables.product.prodname_enterprise %}-Websiteadministrator {% data variables.product.prodname_github_connect %} aktivieren, damit Du beide Umgebungen gleichzeitig durchsuchen kannst. Weitere Informationen findest Du unter „[{% data variables.product.prodname_unified_search %} zwischen {% data variables.product.prodname_enterprise %} und {% data variables.product.prodname_dotcom_the_website %} aktivieren](/enterprise/admin/guides/developer-workflow/enabling-unified-search-between-github-enterprise-server-and-github-com).“
-Sie können beide Umgebungen nur von {% data variables.product.prodname_enterprise %} aus durchsuchen. Um Ihre Suche nach Umgebung einzugrenzen, können Sie eine Filteroption in der {% data variables.search.advanced_url %} oder das Suchpräfix `environment:` verwenden. Um nur nach Inhalten auf {% data variables.product.prodname_enterprise %} zu suchen, verwenden Sie die Suchsyntax `environment:local`. Um nur nach Inhalten auf {% data variables.product.prodname_dotcom_the_website %} zu suchen, verwenden Sie die Suchsyntax `environment:github`.
+Du kannst beide Umgebungen nur von {% data variables.product.prodname_enterprise %} aus durchsuchen. Um Deine Suche nach Umgebung einzugrenzen, kannst Du eine Filteroption in der {% data variables.search.advanced_url %} oder das Suchpräfix `environment:` verwenden. Um nur nach Inhalten auf {% data variables.product.prodname_enterprise %} zu suchen, verwende die Suchsyntax `environment:local`. Um nur nach Inhalten auf {% data variables.product.prodname_dotcom_the_website %} zu suchen, verwende die Suchsyntax `environment:github`.
-Ihr {% data variables.product.prodname_enterprise %}-Websiteadministrator kann {% data variables.product.prodname_unified_search %} für alle öffentlichen Repositorys, alle privaten Repositorys oder nur bestimmte private Repositorys in der verbundenen {% data variables.product.prodname_ghe_cloud %}-Organisation aktivieren.
+Dein {% data variables.product.prodname_enterprise %}-Websiteadministrator kann {% data variables.product.prodname_unified_search %} für alle öffentlichen Repositorys, alle privaten Repositorys oder nur bestimmte private Repositorys in der verbundenen {% data variables.product.prodname_ghe_cloud %}-Organisation aktivieren.
If your site administrator enables
{% data variables.product.prodname_unified_search %} in private repositories, you can only search in the private repositories that the administrator enabled {% data variables.product.prodname_unified_search %} for and that you have access to in the connected {% data variables.product.prodname_dotcom_the_website %} organization. Deine {% data variables.product.prodname_enterprise %}-Administratoren und Organisationsinhaber auf {% data variables.product.prodname_dotcom_the_website %} können keine privaten Repositorys durchsuchen, die Deinem Konto gehören. Um die entsprechenden privaten Repositorys zu durchsuchen, musst Du die Suche auf privaten Repositorys auf Deinen persönlichen Konten auf {% data variables.product.prodname_dotcom_the_website %} und {% data variables.product.prodname_enterprise %} aktivieren. Weitere Informationen findest Du unter „[Die Suche auf privaten {% data variables.product.prodname_dotcom_the_website %}-Repositorys in Deinem {% data variables.product.prodname_enterprise %}-Konto aktivieren](/articles/enabling-private-github-com-repository-search-in-your-github-enterprise-server-account).“
diff --git a/translations/de-DE/content/github/searching-for-information-on-github/searching-code.md b/translations/de-DE/content/github/searching-for-information-on-github/searching-code.md
index d54496c674e2..7cabaf4af6e7 100644
--- a/translations/de-DE/content/github/searching-for-information-on-github/searching-code.md
+++ b/translations/de-DE/content/github/searching-for-information-on-github/searching-code.md
@@ -11,7 +11,7 @@ versions:
github-ae: '*'
---
-Sie können Code global auf {% data variables.product.product_name %} oder in bestimmten Repositorys oder Organisationen durchsuchen. Um den Code aller öffentlichen Repositorys zu durchsuchen, musst Du bei einem {% data variables.product.product_name %}-Konto angemeldet sein. Weitere Informationen findest Du unter „[Informationen zur Suche auf GitHub](/articles/about-searching-on-github).“
+{% data reusables.search.you-can-search-globally %} For more information, see "[About searching on GitHub](/articles/about-searching-on-github)."
Du kannst Code nur mit den diesen Qualifizierern der Codesuche durchsuchen. Spezifische Qualifizierer für Repositorys, Benutzer oder Commits funktionieren bei der Durchsuchung von Code nicht.
@@ -21,16 +21,16 @@ Du kannst Code nur mit den diesen Qualifizierern der Codesuche durchsuchen. Spez
Aufgrund der Komplexität der Codesuche gelten bei der Durchführung der Suche Einschränkungen:
-- {% data reusables.search.required_login %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- {% data reusables.search.required_login %}{% endif %}
- Code in [Forks](/articles/about-forks) ist nur durchsuchbar, wenn für den Fork mehr Sterne vergeben wurden als für das übergeordnete Repository. Forks mit weniger Sternen als das übergeordnete Repository sind **nicht** für die Codesuche indiziert. Um Forks mit mehr Sternen als das übergeordnete Repository in die Suchergebnisse einzuschließen, musst Du Deiner Abfrage `fork:true` oder `fork:only` hinzufügen. Weitere Informationen finden Sie unter „[Forks durchsuchen](/articles/searching-in-forks)“.
- Only the _default branch_ is indexed for code search.{% if currentVersion == "free-pro-team@latest" %}
- Nur Dateien kleiner 384 KB sind durchsuchbar.{% else %}* Nur Dateien kleiner 5 MB sind durchsuchbar.
- Nur die ersten 500 KB jeder Datei sind durchsuchbar.{% endif %}
- Nur Repositorys mit weniger als 500.000 Dateien sind durchsuchbar.
-- Angemeldete Benutzer können alle öffentlichen Repositorys durchsuchen.
-- Deine Quellcode-Suche muss mindestens einen Suchbegriff enthalten, ausgenommen bei [`filename`-Suchen](#search-by-filename). Beispielsweise ist eine Suche nach [`language:javascript`](https://github.com/search?utf8=%E2%9C%93&q=language%3Ajavascript&type=Code&ref=searchresults) ungültig, [`amazing language:javascript`](https://github.com/search?utf8=%E2%9C%93&q=amazing+language%3Ajavascript&type=Code&ref=searchresults) ist dagegen gültig.
+- Ihre Suchabfrage nach Code muss mindestens einen Suchbegriff enthalten. Ausgenommen hiervon sind Suchen nach [`filename`](#search-by-filename). Beispielsweise ist eine Suche nach [`language:javascript`](https://github.com/search?utf8=%E2%9C%93&q=language%3Ajavascript&type=Code&ref=searchresults) ungültig, [`amazing language:javascript`](https://github.com/search?utf8=%E2%9C%93&q=amazing+language%3Ajavascript&type=Code&ref=searchresults) ist dagegen gültig.
- Die Suchergebnisse können maximal zwei gefundene Fragmente der gleichen Datei anzeigen, selbst wenn die Datei mehr Treffer enthält.
-- Folgende Platzhalterzeichen können in Suchabfragen nicht verwendet werden: . , : ; / \ ` ' " = * ! ? # $ & + ^ | ~ < > ( ) { } [ ]
. Diese Zeichen werden bei der Suche schlicht ignoriert.
+- Folgende Platzhalterzeichen können in Suchabfragen nicht verwendet werden: . , : ; / \ ` ' " = * ! ? # $ & + ^ | ~ < > ( ) { } [ ]
. Diese Zeichen werden bei der Suche ignoriert.
### Suche nach Dateiinhalten oder Dateipfad
diff --git a/translations/de-DE/content/github/searching-for-information-on-github/searching-commits.md b/translations/de-DE/content/github/searching-for-information-on-github/searching-commits.md
index 22cefdf85a85..8e30daa5c5be 100644
--- a/translations/de-DE/content/github/searching-for-information-on-github/searching-commits.md
+++ b/translations/de-DE/content/github/searching-for-information-on-github/searching-commits.md
@@ -96,14 +96,11 @@ Wenn Du Commits in allen Repositorys suchst, die einem bestimmten Benutzer oder
| org:ORGNAME
| [**test org:github**](https://github.com/search?utf8=%E2%9C%93&q=test+org%3Agithub&type=Commits) sucht in Repositorys der Organisation @github nach Commits, deren Mitteilungen das Wort „test“ enthalten. |
| repo:USERNAME/REPO
| [**language repo:defunkt/gibberish**](https://github.com/search?utf8=%E2%9C%93&q=language+repo%3Adefunkt%2Fgibberish&type=Commits) sucht im Repository „gibberish“ des Benutzers @defunkt nach Commits, deren Mitteilungen das Wort „language“ enthalten. |
-### Öffentliche oder private Repositorys filtern
+### Filter by repository visibility
-Der Qualifizierer `is` gleicht öffentliche oder private Commits ab.
+The `is` qualifier matches commits from repositories with the specified visibility. Weitere Informationen findest Du unter „[Über Sichtbarkeit von Repositorys](/github/creating-cloning-and-archiving-repositories/about-repository-visibility).
-| Qualifizierer | Beispiel |
-| ------------- | ----------------------------------------------------------------------------------------------------- |
-| `is:public` | [**is:public**](https://github.com/search?q=is%3Apublic&type=Commits) gleicht öffentliche Commits ab. |
-| `is:private` | [**is:public**](https://github.com/search?q=is%3Apublic&type=Commits) gleicht private Commits ab. |
+| Qualifier | Example | ------------- | ------------- |{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %} | `is:public` | [**is:public**](https://github.com/search?q=is%3Apublic&type=Commits) matches commits to public repositories.{% endif %} | `is:internal` | [**is:internal**](https://github.com/search?q=is%3Ainternal&type=Commits) matches commits to internal repositories. | `is:private` | [**is:private**](https://github.com/search?q=is%3Aprivate&type=Commits) matches commits to private repositories.
### Weiterführende Informationen
diff --git a/translations/de-DE/content/github/searching-for-information-on-github/searching-for-repositories.md b/translations/de-DE/content/github/searching-for-information-on-github/searching-for-repositories.md
index 034bb787ef12..993891f0b447 100644
--- a/translations/de-DE/content/github/searching-for-information-on-github/searching-for-repositories.md
+++ b/translations/de-DE/content/github/searching-for-information-on-github/searching-for-repositories.md
@@ -10,7 +10,7 @@ versions:
github-ae: '*'
---
-Sie können Repositorys global auf {% data variables.product.product_name %} oder in einer bestimmten Organisation durchsuchen. Weitere Informationen findest Du unter „[Informationen zur Suche auf {% data variables.product.prodname_dotcom %}](/articles/about-searching-on-github).“
+Sie können Repositorys global auf {% data variables.product.product_location %} oder in einer bestimmten Organisation durchsuchen. Weitere Informationen findest Du unter „[Informationen zur Suche auf {% data variables.product.prodname_dotcom %}](/articles/about-searching-on-github).“
Um Forks in die Suchergebnisse einzuschließen, musst du Deiner Abfrage den Qualifizierer `fork:true` oder `fork:only` hinzufügen. Weitere Informationen finden Sie unter „[Forks durchsuchen](/articles/searching-in-forks)“.
@@ -20,22 +20,22 @@ Um Forks in die Suchergebnisse einzuschließen, musst du Deiner Abfrage den Qual
Mit dem Qualifizierer `in` kannst Du Deine Suche auf den Namen, die Beschreibung oder den Inhalt der README-Dateien der Repositorys oder jede beliebige Kombination derselben eingrenzen. Ohne diesen Qualifizierer werden nur die Namen und Beschreibungen der Repositorys durchsucht.
-| Qualifizierer | Beispiel |
-| ----------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
-| `in:name` | [**jquery in:name**](https://github.com/search?q=jquery+in%3Aname&type=Repositories) sucht Repositorys, deren Namen die Zeichenfolge „jquery“ enthalten. |
-| `in:description` | [**jquery in:name,description**](https://github.com/search?q=jquery+in%3Aname%2Cdescription&type=Repositories) sucht Repositorys, deren Namen oder Beschreibungen die Zeichenfolge „jquery“ enthalten. |
-| `in:readme` | [**jquery in:readme**](https://github.com/search?q=jquery+in%3Areadme&type=Repositories) sucht Repositorys, deren README-Dateien die Zeichenfolge „jquery“ enthalten. |
-| `repo:owner/name` | [**repo:octocat/hello-world**](https://github.com/search?q=repo%3Aoctocat%2Fhello-world) sucht nach einem Repository mit einem bestimmten Namen. |
+| Qualifizierer | Beispiel |
+| ----------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `in:name` | [**jquery in:name**](https://github.com/search?q=jquery+in%3Aname&type=Repositories) matches repositories with "jquery" in the repository name. |
+| `in:description` | [**jquery in:name,description**](https://github.com/search?q=jquery+in%3Aname%2Cdescription&type=Repositories) matches repositories with "jquery" in the repository name or description. |
+| `in:readme` | [**jquery in:readme**](https://github.com/search?q=jquery+in%3Areadme&type=Repositories) matches repositories mentioning "jquery" in the repository's README file. |
+| `repo:owner/name` | [**repo:octocat/hello-world**](https://github.com/search?q=repo%3Aoctocat%2Fhello-world) sucht nach einem Repository mit einem bestimmten Namen. |
### Suche nach Repository-Inhalt
-Mit dem Qualifizierer `in:readme` kannst Du Repositorys nach Inhalten deren README-Dateien suchen.
+You can find a repository by searching for content in the repository's README file using the `in:readme` qualifier. Weitere Informationen finden Sie unter „[Informationen zu README-Dateien](/github/creating-cloning-and-archiving-repositories/about-readmes)“.
`in:readme` ist die einzige Möglichkeit, Repositorys anhand bestimmter Inhalte im Repository zu finden. Wenn Du nach einer bestimmten Datei oder einem bestimmten Inhalt innerhalb eines Repositorys suchst, verwende den Dateifinder oder die code-spezifischen Suchbegriffe. Weitere Informationen findest Du in den Abschnitten „[Dateien auf {% data variables.product.prodname_dotcom %} finden](/articles/finding-files-on-github)“ und „[Code durchsuchen](/articles/searching-code).“
-| Qualifizierer | Beispiel |
-| ------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
-| `in:readme` | [**octocat in:readme**](https://github.com/search?q=octocat+in%3Areadme&type=Repositories) sucht Repositorys, deren README-Dateien die Zeichenfolge „octocat“ enthalten. |
+| Qualifizierer | Beispiel |
+| ------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `in:readme` | [**octocat in:readme**](https://github.com/search?q=octocat+in%3Areadme&type=Repositories) matches repositories mentioning "octocat" in the repository's README file. |
### Suche innerhalb der Repositorys eines Benutzers oder einer Organisation
@@ -48,7 +48,7 @@ Wenn Du alle Repositorys durchsuchen möchtest, die einem bestimmten Benutzer od
### Suche nach Repository-Größe
-Der Qualifizierer `size` sucht Repositorys mit einer bestimmten Größe (in Kilobyte). Zur Angabe des Größenbereichs verwende die [„Größer als“-, „Kleiner als“- oder Bereichsqualifizierer](/articles/understanding-the-search-syntax).
+The `size` qualifier finds repositories that match a certain size (in kilobytes), using greater than, less than, and range qualifiers. For more information, see "[Understanding the search syntax](/github/searching-for-information-on-github/understanding-the-search-syntax)."
| Qualifizierer | Beispiel |
| ------------------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -59,7 +59,7 @@ Der Qualifizierer `size` sucht Repositorys mit einer bestimmten Größe (in Kilo
### Suche nach Anzahl der Follower
-Mit dem Qualifizierer `followers` kannst Du Repositorys nach der Anzahl ihrer Follower filtern. Zur Angabe der Anzahl der Follower verwende [„Größer als“-, „Kleiner als“- oder Bereichsqualifizierer](/articles/understanding-the-search-syntax).
+You can filter repositories based on the number of users who follow the repositories, using the `followers` qualifier with greater than, less than, and range qualifiers. For more information, see "[Understanding the search syntax](/github/searching-for-information-on-github/understanding-the-search-syntax)."
| Qualifizierer | Beispiel |
| ------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -68,7 +68,7 @@ Mit dem Qualifizierer `followers` kannst Du Repositorys nach der Anzahl ihrer Fo
### Suche nach Anzahl der Forks
-Mit dem Qualifizierer `forks` kannst Du Repositorys nach der Anzahl ihrer Forks filtern. Zur Angabe der Anzahl der Forks verwende [„Größer als“-, „Kleiner als“- oder Bereichsqualifizierer](/articles/understanding-the-search-syntax).
+The `forks` qualifier specifies the number of forks a repository should have, using greater than, less than, and range qualifiers. For more information, see "[Understanding the search syntax](/github/searching-for-information-on-github/understanding-the-search-syntax)."
| Qualifizierer | Beispiel |
| ------------------------- | --------------------------------------------------------------------------------------------------------------------------------------- |
@@ -79,7 +79,7 @@ Mit dem Qualifizierer `forks` kannst Du Repositorys nach der Anzahl ihrer Forks
### Suche nach Anzahl der Sterne
-Du kannst Repositorys nach der Anzahl Ihrer [Sterne](/articles/saving-repositories-with-stars) filtern. Zur Angabe der Anzahl der Sterne verwende [„Größer als“-, „Kleiner als“- oder Bereichsqualifizierer](/articles/understanding-the-search-syntax)
+You can search repositories based on the number of stars the repositories have, using greater than, less than, and range qualifiers. For more information, see "[Saving repositories with stars](/github/getting-started-with-github/saving-repositories-with-stars)" and "[Understanding the search syntax](/github/searching-for-information-on-github/understanding-the-search-syntax)."
| Qualifizierer | Beispiel |
| ------------------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -103,7 +103,7 @@ Beide Qualifizierer verwenden als Parameter ein Datum. {% data reusables.time_da
### Suche nach Sprache
-Du kannst Repositorys nach der primären Programmiersprache suchen, in der sie geschrieben wurden.
+You can search repositories based on the language of the code in the repositories.
| Qualifizierer | Beispiel |
| ------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
@@ -111,7 +111,7 @@ Du kannst Repositorys nach der primären Programmiersprache suchen, in der sie g
### Suche nach Thema
-Du kannst nach allen Repositorys suchen, die durch ein bestimmtes [Thema](/articles/classifying-your-repository-with-topics) klassifiziert wurden.
+You can find all of the repositories that are classified with a particular topic. Weitere Informationen finden Sie unter „[Repository mit Themen klassifizieren](/github/administering-a-repository/classifying-your-repository-with-topics)“.
| Qualifizierer | Beispiel |
| ------------------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -119,53 +119,55 @@ Du kannst nach allen Repositorys suchen, die durch ein bestimmtes [Thema](/artic
### Suche nach Anzahl der Themen
-Mit dem Qualifizierer `topics` kannst Du Repositorys nach der Anzahl der [Themen](/articles/classifying-your-repository-with-topics) filtern, durch die sie klassifiziert wurden. Zur Angabe der Anzahl der Themen verwende die [„Größer als“-, „Kleiner als“- oder Bereichsqualifizierer](/articles/understanding-the-search-syntax).
+You can search repositories by the number of topics that have been applied to the repositories, using the `topics` qualifier along with greater than, less than, and range qualifiers. For more information, see "[Classifying your repository with topics](/github/administering-a-repository/classifying-your-repository-with-topics)" and "[Understanding the search syntax](/github/searching-for-information-on-github/understanding-the-search-syntax)."
| Qualifizierer | Beispiel |
| -------------------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------- |
| topics:n
| [**topics:5**](https://github.com/search?utf8=%E2%9C%93&q=topics%3A5&type=Repositories&ref=searchresults) sucht Repositorys mit fünf Themen. |
| | [**topics:>3**](https://github.com/search?utf8=%E2%9C%93&q=topics%3A%3E3&type=Repositories&ref=searchresults) sucht Repositorys mit mehr als drei Themen. |
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
### Suche nach Lizenz
-Du kannst Repositorys nach ihrer [Lizenz](/articles/licensing-a-repository) filtern. Zum Filtern von Repositorys nach einer bestimmten Lizenz oder Lizenzfamilie musst Du ein [Lizenzstichwort](/articles/licensing-a-repository/#searching-github-by-license-type) angeben.
+You can search repositories by the type of license in the repositories. You must use a license keyword to filter repositories by a particular license or license family. Weitere Informationen finden Sie unter „[Ein Repository lizenzieren](/github/creating-cloning-and-archiving-repositories/licensing-a-repository)“.
| Qualifizierer | Beispiel |
| -------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| license:LICENSE_KEYWORD
| [**license:apache-2.0**](https://github.com/search?utf8=%E2%9C%93&q=license%3Aapache-2.0&type=Repositories&ref=searchresults) sucht Repositorys, die unter Apache License 2.0 lizenziert sind. |
-### Suche nach öffentlichen oder privaten Repositorys
+{% endif %}
+
+### Search by repository visibility
-Du kannst Deine Suche danach filtern, ob ein Repository öffentlich oder privat ist.
+You can filter your search based on the visibility of the repositories. Weitere Informationen findest Du unter „[Über Sichtbarkeit von Repositorys](/github/creating-cloning-and-archiving-repositories/about-repository-visibility)."
-| Qualifizierer | Beispiel |
-| ------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `is:public` | [**is:public org:github**](https://github.com/search?q=is%3Apublic+org%3Agithub&type=Repositories&utf8=%E2%9C%93) sucht öffentliche GitHub-Repositorys. |
-| `is:private` | [**is:private pages**](https://github.com/search?utf8=%E2%9C%93&q=pages+is%3Aprivate&type=Repositories) sucht private Repositorys, auf die Du Zugriff hast und die das Wort „pages“ enthalten. |
+| Qualifier | Example | ------------- | ------------- |{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %} | `is:public` | [**is:public org:github**](https://github.com/search?q=is%3Apublic+org%3Agithub&type=Repositories) matches public repositories owned by {% data variables.product.company_short %}.{% endif %} | `is:internal` | [**is:internal test**](https://github.com/search?q=is%3Ainternal+test&type=Repositories) matches internal repositories that you can access and contain the word "test". | `is:private` | [**is:private pages**](https://github.com/search?q=is%3Aprivate+pages&type=Repositories) matches private repositories that you can access and contain the word "pages."
{% if currentVersion == "free-pro-team@latest" %}
### Suche auf Basis der Spiegelung eines Repositorys
-Du kannst Repositorys danach durchsuchen, ob sie ein Spiegel sind und an anderer Stelle gehostet werden. Weitere Informationen findest du unter „[Möglichkeiten finden, Beiträge an Open-Source auf {% data variables.product.prodname_dotcom %} zu leisten](/github/getting-started-with-github/finding-ways-to-contribute-to-open-source-on-github)."
+You can search repositories based on whether the repositories are mirrors and hosted elsewhere. Weitere Informationen findest du unter „[Möglichkeiten finden, Beiträge an Open-Source auf {% data variables.product.prodname_dotcom %} zu leisten](/github/getting-started-with-github/finding-ways-to-contribute-to-open-source-on-github)."
-| Qualifizierer | Beispiel |
-| -------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `mirror:true` | [**mirror:true GNOME**](https://github.com/search?utf8=%E2%9C%93&q=mirror%3Atrue+GNOME&type=) sucht gespiegelte Repositorys, die das Wort „GNOME“ enthalten. |
-| `mirror:false` | [**mirror:false GNOME**](https://github.com/search?utf8=%E2%9C%93&q=mirror%3Afalse+GNOME&type=) sucht nicht gespiegelte Repositorys, die das Wort „GNOME“ enthalten. |
+| Qualifizierer | Beispiel |
+| -------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `mirror:true` | [**mirror:true GNOME**](https://github.com/search?utf8=%E2%9C%93&q=mirror%3Atrue+GNOME&type=) matches repositories that are mirrors and contain the word "GNOME." |
+| `mirror:false` | [**mirror:false GNOME**](https://github.com/search?utf8=%E2%9C%93&q=mirror%3Afalse+GNOME&type=) matches repositories that are not mirrors and contain the word "GNOME." |
{% endif %}
### Suche auf Basis der Archivierung eines Repositorys
-Bei der Suche kannst Du Repositorys auf Basis dessen filtern, ob sie [archiviert](/articles/about-archiving-repositories) sind.
+You can search repositories based on whether or not the repositories are archived. For more information, see "[About archiving repositories](/github/creating-cloning-and-archiving-repositories/about-archiving-repositories)."
-| Qualifizierer | Beispiel |
-| ---------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
-| `archived:true` | [**archived:true GNOME**](https://github.com/search?utf8=%E2%9C%93&q=archived%3Atrue+GNOME&type=) sucht archivierte Repositorys, die das Wort „GNOME“ enthalten. |
-| `archived:false` | [**archived:false GNOME**](https://github.com/search?utf8=%E2%9C%93&q=archived%3Afalse+GNOME&type=) sucht nicht archivierte Repositorys, die das Wort „GNOME“ enthalten. |
+| Qualifizierer | Beispiel |
+| ---------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `archived:true` | [**archived:true GNOME**](https://github.com/search?utf8=%E2%9C%93&q=archived%3Atrue+GNOME&type=) matches repositories that are archived and contain the word "GNOME." |
+| `archived:false` | [**archived:false GNOME**](https://github.com/search?utf8=%E2%9C%93&q=archived%3Afalse+GNOME&type=) matches repositories that are not archived and contain the word "GNOME." |
{% if currentVersion == "free-pro-team@latest" %}
+
### Suche nach Anzahl der Issues mit der Kennzeichnung `good first issue` oder `help wanted`
Mit den Qualifizierern `good-first-issues:>n` und `help-wanted-issues:>n` kannst Du Repositorys mit einer Mindestanzahl an Issues mit der Kennzeichnung `good-first-issue` oder `help-wanted` suchen. Weitere Informationen findest Du auf „[Ermutigen hilfreicher Beiträge zu Deinem Projekt über Kennzeichnungen](/github/building-a-strong-community/encouraging-helpful-contributions-to-your-project-with-labels)."
@@ -174,6 +176,7 @@ Mit den Qualifizierern `good-first-issues:>n` und `help-wanted-issues:>n` kannst
| -------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| `good-first-issues:>n` | [**good-first-issues:>2 javascript**](https://github.com/search?utf8=%E2%9C%93&q=javascript+good-first-issues%3A%3E2&type=) sucht Repositorys mit mehr als zwei Issues mit der Kennzeichnung `good-first-issue`, die das Wort „javascript“ enthalten. |
| `help-wanted-issues:>n` | [**help-wanted-issues:>4 react**](https://github.com/search?utf8=%E2%9C%93&q=react+help-wanted-issues%3A%3E4&type=) sucht Repositorys mit mehr als vier Issues mit der Kennzeichnung `help-wanted`, die das Wort „React“ enthalten. |
+
{% endif %}
### Weiterführende Informationen
diff --git a/translations/de-DE/content/github/searching-for-information-on-github/searching-issues-and-pull-requests.md b/translations/de-DE/content/github/searching-for-information-on-github/searching-issues-and-pull-requests.md
index 140baf2d46ec..9ef710d922ec 100644
--- a/translations/de-DE/content/github/searching-for-information-on-github/searching-issues-and-pull-requests.md
+++ b/translations/de-DE/content/github/searching-for-information-on-github/searching-issues-and-pull-requests.md
@@ -64,66 +64,63 @@ Mit dem Qualifizierer `state` oder `is` kannst Du Issues und Pull Requests danac
| `is:open` | [**performance is:open is:issue**](https://github.com/search?q=performance+is%3Aopen+is%3Aissue&type=Issues) sucht offene Issues, die das Wort „performance“ enthalten. |
| `is:closed` | [**android is:closed**](https://github.com/search?utf8=%E2%9C%93&q=android+is%3Aclosed&type=) sucht geschlossene Issues und Pull Requests, die das Wort „android“ enthalten. |
-### Suche nach öffentlichen oder privaten Repositorys
+### Filter by repository visibility
-Wenn Sie [{% data variables.product.product_name %} vollständig durchsuchen](https://github.com/search), ist eine Filterung der Ergebnisse nach öffentlichen oder privaten Repositorys oft sehr nützlich. Hierzu verwendest Du die Qualifizierer `is:public` und `is:private`.
+You can filter by the visibility of the repository containing the issues and pull requests using the `is` qualifier. Weitere Informationen findest Du unter „[Über Sichtbarkeit von Repositorys](/github/creating-cloning-and-archiving-repositories/about-repository-visibility)."
-| Qualifizierer | Beispiel |
-| ------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `is:public` | [**is:public**](https://github.com/search?q=is%3Apublic&type=Issues) sucht Issues und Pull Requests in allen öffentlichen Repositorys. |
-| `is:private` | [**is:private cupcake**](https://github.com/search?q=is%3Aprivate&type=Issues) sucht Issues und Pull Requests mit dem Wort „cupcake“ in allen privaten Repositorys, auf die Du Zugriff hast. |
+| Qualifier | Example | ------------- | ------------- |{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %} | `is:public` | [**is:public**](https://github.com/search?q=is%3Apublic&type=Issues) matches issues and pull requests in public repositories.{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.20" or currentVersion == "github-ae@latest" %} | `is:internal` | [**is:internal**](https://github.com/search?q=is%3Ainternal&type=Issues) matches issues and pull requests in internal repositories.{% endif %} | `is:private` | [**is:private cupcake**](https://github.com/search?q=is%3Aprivate+cupcake&type=Issues) matches issues and pull requests that contain the word "cupcake" in private repositories you can access.
### Suche nach Autor
Der Qualifizierer `author` sucht Issues und Pull Requests, die von einem bestimmten Benutzer oder Integrationskonto erstellt wurden.
-| Qualifizierer | Beispiel |
-| ------------------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| author:USERNAME
| [**cool author:gjtorikian**](https://github.com/search?q=cool+author%3Agjtorikian&type=Issues) sucht von @gjtorikian erstellte Issues und Pull Requests, die das Wort „cool“ enthalten. |
-| | [**bootstrap in:body author:mdo**](https://github.com/search?q=bootstrap+in%3Abody+author%3Amdo&type=Issues) sucht von @mdo verfasste Issues, die im Textteil das Wort „bootstrap“ enthalten. |
-| author:app/USERNAME
| [**author:app/robot**](https://github.com/search?q=author%3Aapp%2Frobot&type=Issues) sucht Issues, die vom Integrationskonto „robot“ erstellt wurden. |
+| Qualifizierer | Beispiel |
+| ------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| author:USERNAME
| [**cool author:gjtorikian**](https://github.com/search?q=cool+author%3Agjtorikian&type=Issues) matches issues and pull requests with the word "cool" that were created by @gjtorikian. |
+| | [**bootstrap in:body author:mdo**](https://github.com/search?q=bootstrap+in%3Abody+author%3Amdo&type=Issues) matches issues written by @mdo that contain the word "bootstrap" in the body. |
+| author:app/USERNAME
| [**author:app/robot**](https://github.com/search?q=author%3Aapp%2Frobot&type=Issues) matches issues created by the integration account named "robot." |
### Suche nach Bearbeiter
Der Qualifizierer `assignee` sucht Issues und Pull Requests, die einem bestimmten Benutzer zugewiesen sind. Nach Issues und Pull Requests mit _beliebigem_ Bearbeiter kannst Du nicht suchen. Du kannst jedoch nach [Issues und Pull Requests suchen, denen kein Bearbeiter zugewiesen ist](#search-by-missing-metadata).
-| Qualifizierer | Beispiel |
-| ------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| assignee:USERNAME
| [**assignee:vmg repo:libgit2/libgit2**](https://github.com/search?utf8=%E2%9C%93&q=assignee%3Avmg+repo%3Alibgit2%2Flibgit2&type=Issues) sucht Issues und Pull Requests im Projekt „libgit2“ von libgit2, die @vmg zugewiesen sind. |
+| Qualifizierer | Beispiel |
+| ------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| assignee:USERNAME
| [**assignee:vmg repo:libgit2/libgit2**](https://github.com/search?utf8=%E2%9C%93&q=assignee%3Avmg+repo%3Alibgit2%2Flibgit2&type=Issues) matches issues and pull requests in libgit2's project libgit2 that are assigned to @vmg. |
### Suche nach Erwähnung
Der Qualifizierer `mentions` sucht Issues, in denen ein bestimmter Benutzer erwähnt wird. Weitere Informationen findest Du unter „[Personen und Teams erwähnen](/articles/basic-writing-and-formatting-syntax/#mentioning-people-and-teams).“
-| Qualifizierer | Beispiel |
-| ------------------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| mentions:USERNAME
| [**resque mentions:defunkt**](https://github.com/search?q=resque+mentions%3Adefunkt&type=Issues) sucht Issues, die das Wort „resque“ enthalten und „@defunkt“ erwähnen. |
+| Qualifizierer | Beispiel |
+| ------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| mentions:USERNAME
| [**resque mentions:defunkt**](https://github.com/search?q=resque+mentions%3Adefunkt&type=Issues) matches issues with the word "resque" that mention @defunkt. |
### Suche nach Teamerwähnung
Mit dem Qualifizierer `team` kannst du innerhalb von Organisationen und Teams, zu denen Du gehörst, Issues oder Pull Requests suchen, die ein bestimmtes Team innerhalb der Organisation @erwähnen. Ersetze in den folgenden Beispielen die Namen durch den Namen Deiner Organisation und Deines Teams, um eine Suche durchzuführen.
-| Qualifizierer | Beispiel |
-| ------------------------- | --------------------------------------------------------------------------------------------------------- |
-| team:ORGNAME/TEAMNAME
| **team:jekyll/owners** sucht Issues, in denen das Team `@jekyll/owners` erwähnt wird. |
-| | **team:myorg/ops is:open is:pr** sucht offene Pull Requests, in denen das Team `@myorg/ops` erwähnt wird. |
+| Qualifizierer | Beispiel |
+| ------------------------- | ----------------------------------------------------------------------------------------------------- |
+| team:ORGNAME/TEAMNAME
| **team:jekyll/owners** matches issues where the `@jekyll/owners` team is mentioned. |
+| | **team:myorg/ops is:open is:pr** matches open pull requests where the `@myorg/ops` team is mentioned. |
### Suche nach Kommentierer
Der Qualifizierer `commenter` sucht Issues, die einen Kommentar eines bestimmten Benutzers enthalten.
-| Qualifizierer | Beispiel |
-| ------------------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| commenter:USERNAME
| [**github commenter:defunkt org:github**](https://github.com/search?utf8=%E2%9C%93&q=github+commenter%3Adefunkt+org%3Agithub&type=Issues) sucht Issues in Repositorys von GitHub, die das Wort „github“ enthalten und von Benutzer @defunkt kommentiert wurden. |
+| Qualifizierer | Beispiel |
+| ------------------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| commenter:USERNAME
| [**github commenter:defunkt org:github**](https://github.com/search?utf8=%E2%9C%93&q=github+commenter%3Adefunkt+org%3Agithub&type=Issues) matches issues in repositories owned by GitHub, that contain the word "github," and have a comment by @defunkt. |
### Suche nach beteiligtem Benutzer
Mit dem Qualifizierer `involves` kannst Du Issues suchen, an denen auf die eine oder andere Weise ein bestimmter Benutzer beteiligt ist. Der Qualifizierer `involves` ist ein logisches ODER zwischen den Qualifizierern `author`, `assignee`, `mentions` und `commenter` für einen einzelnen Benutzer. Dieser Qualifizierer sucht also Issues und Pull Requests, die von einem bestimmten Benutzer erstellt wurden, diesem zugewiesen sind, diesen erwähnen oder in denen dieser Benutzer einen Kommentar hinterlassen hat.
-| Qualifizierer | Beispiel |
-| ------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| involves:USERNAME
| **[involves:defunkt involves:jlord](https://github.com/search?q=involves%3Adefunkt+involves%3Ajlord&type=Issues)** sucht Issues, an denen entweder @defunkt oder @jlord beteiligt ist. |
-| | [**NOT bootstrap in:body involves:mdo**](https://github.com/search?q=NOT+bootstrap+in%3Abody+involves%3Amdo&type=Issues) sucht Issues, an denen @mdo beteiligt ist, die im Textteil jedoch nicht das Wort „bootstrap“ enthalten. |
+| Qualifizierer | Beispiel |
+| ------------------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| involves:USERNAME
| **[involves:defunkt involves:jlord](https://github.com/search?q=involves%3Adefunkt+involves%3Ajlord&type=Issues)** matches issues either @defunkt or @jlord are involved in. |
+| | [**NOT bootstrap in:body involves:mdo**](https://github.com/search?q=NOT+bootstrap+in%3Abody+involves%3Amdo&type=Issues) matches issues @mdo is involved in that do not contain the word "bootstrap" in the body. |
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.20" or currentVersion == "github-ae@latest" %}
### Suche nach verknüpften Issues und Pull Request
@@ -138,7 +135,7 @@ Du kannst Deine Ergebnisse auf Issues einschränken, die mit einem Pull-Request
### Suche nach Kennzeichnung
-Mit dem Qualifizierer `label` kannst Du Deine Suchergebnisse nach Kennzeichnungen eingrenzen. Da Issues verschiedene Kennzeichnungen aufweisen können, kannst du für jeden Issue einen eigenen Qualifizierer einsetzen.
+Mit dem Kennzeichner `label` können Sie Ihre Suchergebnisse nach Kennzeichnungen eingrenzen. Da Issues verschiedene Kennzeichnungen aufweisen können, können Sie für jeden Issue einen eigenen Kennzeichner auflisten.
| Qualifizierer | Beispiel |
| -------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -148,7 +145,7 @@ Mit dem Qualifizierer `label` kannst Du Deine Suchergebnisse nach Kennzeichnunge
### Suche nach Meilenstein
-Der Qualifizierer `milestone` findet Issues oder Pull Requests, die innerhalb eines Repositorys Teil eines [Meilensteins](/articles/about-milestones) sind.
+Der Kennzeichner `milestone` sucht Issues oder Pull Requests, die innerhalb eines Repositorys Teil eines [Meilensteins](/articles/about-milestones) sind.
| Qualifizierer | Beispiel |
| -------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -157,7 +154,7 @@ Der Qualifizierer `milestone` findet Issues oder Pull Requests, die innerhalb ei
### Suche nach Projektboard
-Mit dem Qualifizierer <`project` kannst Du Issues suchen, die innerhalb eines Repositorys oder einer Organisation einem bestimmten [Projektboard](/articles/about-project-boards/) zugeordnet sind. Projektboards werden anhand ihrer Projektboardnummer gesucht. Die Nummer eines Projektboards befindet sich am Ende seiner URL.
+Mit dem Kennzeichner `project` können Sie Issues suchen, die innerhalb eines Repositorys oder einer Organisation einem bestimmten [Projektboard](/articles/about-project-boards/) zugeordnet sind. Projektboards werden anhand ihrer Projektboardnummer gesucht. Die Nummer eines Projektboards befindet sich am Ende von dessen URL.
| Qualifizierer | Beispiel |
| -------------------------- | -------------------------------------------------------------------------------------------------------------------- |
@@ -166,7 +163,7 @@ Mit dem Qualifizierer <`project` kannst Du Issues suchen, die innerhalb eines Re
### Suche nach Commit-Status
-Du kannst Pull Requests nach dem Status ihrer Commits filtern. Dieser Filter ist besonders nützlich, wenn Sie die [Status-API](/v3/repos/statuses/) oder einen CI-Dienst verwenden.
+Sie können Pull Requests nach dem Status ihrer Commits filtern. Dieser Filter ist besonders nützlich, wenn Sie die [Status-API](/v3/repos/statuses/) oder einen CI-Dienst verwenden.
| Qualifizierer | Beispiel |
| ---------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -176,7 +173,7 @@ Du kannst Pull Requests nach dem Status ihrer Commits filtern. Dieser Filter ist
### Suche nach Commit-SHA
-Wenn Du den spezifischen SHA-Hash eines Commits kennst, kannst Du ihn für die Suche nach Pull Requests verwenden, die diesen SHA enthalten. Die SHA-Syntax besteht aus mindestens sieben Zeichen.
+Wenn Sie den spezifischen SHA-Hash eines Commits kennen, können Sie ihn für die Suche nach Pull Requests verwenden, die diesen SHA enthalten. Die SHA-Syntax besteht aus mindestens sieben Zeichen.
| Qualifizierer | Beispiel |
| -------------------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -185,7 +182,7 @@ Wenn Du den spezifischen SHA-Hash eines Commits kennst, kannst Du ihn für die S
### Suche nach Branch-Name
-Du kannst Pull Requests nach dem Branch filtern, aus dem sie stammen (Head-Branch) oder in den sie zusammengeführt werden (Basis-Branch).
+Sie können Pull Requests nach dem Branch filtern, aus dem sie stammen (Head-Branch) oder in den sie gemergt werden (Basis-Branch).
| Qualifizierer | Beispiel |
| -------------------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -194,7 +191,7 @@ Du kannst Pull Requests nach dem Branch filtern, aus dem sie stammen (Head-Branc
### Suche nach Sprache
-Mit dem Qualifizierer `language` kannst Du Issues und Pull Requests in Repositorys suchen, die in einer bestimmten Programmiersprache geschrieben sind.
+Mit dem Kennzeichner `language` können Sie Issues und Pull Requests in Repositorys suchen, die in einer bestimmten Programmiersprache geschrieben sind.
| Qualifizierer | Beispiel |
| -------------------------- | --------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -202,7 +199,7 @@ Mit dem Qualifizierer `language` kannst Du Issues und Pull Requests in Repositor
### Suche nach Anzahl der Kommentare
-Mit dem Qualifizierer `comments` in Verbindung mit [„Größer-als“-, „Kleiner-als“- oder Bereichsqualifizierern](/articles/understanding-the-search-syntax) kannst Du nach der Anzahl der Kommentare filtern.
+Mit dem Kennzeichner `comments` in Verbindung mit dem [„Größer-als“-, „Kleiner-als“- oder dem Bereichskennzeichner](/articles/understanding-the-search-syntax) können Sie nach der Anzahl der Kommentare filtern.
| Qualifizierer | Beispiel |
| -------------------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -211,7 +208,7 @@ Mit dem Qualifizierer `comments` in Verbindung mit [„Größer-als“-, „Klei
### Suche nach Anzahl der Interaktionen
-Mit dem Qualifizierer `interactions` kannst Du Issues und Pull Requests nach der Anzahl ihrer Interaktionen filtern. Zur Angabe der Anzahl der Interaktionen verwende [„Größer-als“-, „Kleiner-als“- oder Bereichsqualifizierer](/articles/understanding-the-search-syntax). Der Interaktionszähler ist die Anzahl Reaktionen und Kommentare zu einem Issue oder Pull Request.
+Mit dem Kennzeichner `interactions` können Sie Issues und Pull Requests nach der Anzahl ihrer Interaktionen filtern. Zur Angabe der Anzahl der Interaktionen verwenden Sie den [„Größer-als“-, „Kleiner-als“- oder den Bereichskennzeichner](/articles/understanding-the-search-syntax). Interaktionen sind Reaktionen und Kommentare zu einem Issue oder Pull Request.
| Qualifizierer | Beispiel |
| -------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------ |
@@ -220,7 +217,7 @@ Mit dem Qualifizierer `interactions` kannst Du Issues und Pull Requests nach der
### Suche nach Anzahl der Reaktionen
-Mit dem Qualifizierer `reactions` kannst Du Issues und Pull Requests nach der Anzahl ihrer Reaktionen filtern. Zur Angabe der Anzahl der Reaktionen verwende [„Größer-als“-, „Kleiner-als“- oder Bereichsqualifizierer](/articles/understanding-the-search-syntax).
+Mit dem Kennzeichner `reactions` können Sie Issues und Pull Requests nach der Anzahl ihrer Reaktionen filtern. Zur Angabe der Anzahl der Reaktionen verwenden Sie den [„Größer-als“-, „Kleiner-als“- oder den Bereichskennzeichner](/articles/understanding-the-search-syntax).
| Qualifizierer | Beispiel |
| -------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -228,7 +225,7 @@ Mit dem Qualifizierer `reactions` kannst Du Issues und Pull Requests nach der An
| | [**reactions:500..1000**](https://github.com/search?q=reactions%3A500..1000) sucht Issues mit 500 bis 1.000 Reaktionen. |
### Suche nach Pull-Request-Entwürfen
-Du kannst nach Pull-Request-Entwürfen suchen. Weitere Informationen findest Du unter „[Informationen zu Pull Requests](/articles/about-pull-requests#draft-pull-requests).“
+Sie können nach Pull-Request-Entwürfen suchen. Weitere Informationen findest Du unter „[Informationen zu Pull Requests](/articles/about-pull-requests#draft-pull-requests).“
| Qualifier | Example | ------------- | -------------{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.20" or currentVersion == "github-ae@latest" %} | `draft:true` | [**draft:true**](https://github.com/search?q=draft%3Atrue) matches draft pull requests. | `draft:false` | [**draft:false**](https://github.com/search?q=draft%3Afalse) findet Pull Requests, die bereit sind für den Review.{% else %} | `is:draft` | [**is:draft**](https://github.com/search?q=is%3Adraft) findet Pull-Request-Entwürfe.{% endif %}
@@ -248,7 +245,7 @@ Du kannst Pull Requests nach ihrem [Review-Status](/articles/about-pull-request-
### Suche nach dem Datum der Erstellung oder letzten Änderung eines Issues oder Pull Requests
-Du kannst Issues nach dem Zeitpunkt der Erstellung oder letzten Änderung filtern. Für die Suche nach dem Erstellungsdatum verwende den Qualifizierer `created`, für die Suche nach dem letzten Änderungsdatum den Qualifizierer `updated`.
+Sie können Issues nach dem Zeitpunkt der Erstellung oder letzten Änderung filtern. Für die Suche nach dem Erstellungsdatum verwenden Sie den Kennzeichner `created`, für die Suche nach dem letzten Änderungsdatum den Kennzeichner `updated`.
Beide Qualifizierer verwenden als Parameter ein Datum. {% data reusables.time_date.date_format %} {% data reusables.time_date.time_format %}
@@ -261,7 +258,7 @@ Beide Qualifizierer verwenden als Parameter ein Datum. {% data reusables.time_da
### Suche nach dem Datum der Schließung eines Issues oder Pull Requests
-Mit dem Qualifizierer `closed` kannst Du Issues und Pull Requests nach dem Datum der Schließung filtern.
+Mit dem Kennzeichner `closed` können Sie Issues und Pull Requests nach ihrem Schließungsdatum filtern.
Der Kennzeichner verwendet als Parameter ein Datum. {% data reusables.time_date.date_format %} {% data reusables.time_date.time_format %}
@@ -274,7 +271,7 @@ Der Kennzeichner verwendet als Parameter ein Datum. {% data reusables.time_date.
### Suche nach dem Merge-Datum eines Issues oder Pull Requests
-Mit dem Qualifizierer `merged` kannst Du Pull Requests nach ihrem Merge-Datum filtern.
+Mit dem Kennzeichner `merged` können Sie Pull Requests nach ihrem Merge-Datum filtern.
Der Kennzeichner verwendet als Parameter ein Datum. {% data reusables.time_date.date_format %} {% data reusables.time_date.time_format %}
@@ -287,7 +284,7 @@ Der Kennzeichner verwendet als Parameter ein Datum. {% data reusables.time_date.
### Suche nach dem Merge-Status eines Pull Requests
-Mit dem Qualifizierer `is` kannst Du Pull Requests danach filtern, ob sie zusammengeführt sind oder nicht.
+Mit dem Kennzeichner `is` können Sie Pull Requests danach filtern, ob sie gemergt oder ungemergt sind.
| Qualifizierer | Beispiel |
| ------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -296,7 +293,7 @@ Mit dem Qualifizierer `is` kannst Du Pull Requests danach filtern, ob sie zusamm
### Suche auf Basis der Archivierung eines Repositorys
-Der Qualifizierer `archived` filtert Suchergebnisse danach, ob sich ein Issue oder Pull Request in einem archivierten Repository befindet.
+Der Kennzeichner `archived` filtert Suchergebnisse danach, ob sich ein Issue oder Pull Request in einem archivierten Repository befindet.
| Qualifizierer | Beispiel |
| ---------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
@@ -305,7 +302,7 @@ Der Qualifizierer `archived` filtert Suchergebnisse danach, ob sich ein Issue od
### Suche nach dem Sperrstatus einer Unterhaltung
-Mit dem Qualifizierer `is` kannst Du Issues oder Pull Requests mit gesperrten Unterhaltungen suchen. Weitere Informationen findest Du unter „[Unterhaltungen sperren](/articles/locking-conversations).“
+Mit dem Kennzeichner `is` können Sie Issues oder Pull Requests mit gesperrten Unterhaltungen suchen. Weitere Informationen finden Sie unter „[Unterhaltungen sperren](/articles/locking-conversations)“.
| Qualifizierer | Beispiel |
| ------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -314,7 +311,7 @@ Mit dem Qualifizierer `is` kannst Du Issues oder Pull Requests mit gesperrten Un
### Suche nach fehlenden Metadaten
-Mit dem Qualifizierer `no` kannst Du Deine Suche auf Issues und Pull Requests eingrenzen, in denen bestimmte Metadaten fehlen. Hierbei kannst Du nach folgenden fehlenden Metadaten suchen:
+Mit dem Kennzeichner `no` können Sie Ihre Suche auf Issues und Pull Requests eingrenzen, in denen bestimmte Metadaten fehlen. Hierbei können Sie nach folgenden fehlenden Metadaten suchen:
* Kennzeichnungen
* Meilensteine
diff --git a/translations/de-DE/content/github/setting-up-and-managing-organizations-and-teams/about-your-organization-dashboard.md b/translations/de-DE/content/github/setting-up-and-managing-organizations-and-teams/about-your-organization-dashboard.md
index 07be5d94c111..240718f5747f 100644
--- a/translations/de-DE/content/github/setting-up-and-managing-organizations-and-teams/about-your-organization-dashboard.md
+++ b/translations/de-DE/content/github/setting-up-and-managing-organizations-and-teams/about-your-organization-dashboard.md
@@ -37,8 +37,8 @@ Beispielsweise werden im Newsfeed der Organisation Aktualisierungen angezeigt, w
- einen Pull-Request-Review-Kommentar absendet,
- ein Repository forkt,
- eine Wiki-Seite erstellt,
- - Commits pusht,
- - ein öffentliches Repository erstellt.
+ - Pushes commits.{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+ - Creates a public repository.{% endif %}
### Weiterführende Informationen
diff --git a/translations/de-DE/content/github/setting-up-and-managing-organizations-and-teams/restricting-repository-visibility-changes-in-your-organization.md b/translations/de-DE/content/github/setting-up-and-managing-organizations-and-teams/restricting-repository-visibility-changes-in-your-organization.md
index 41bb87dc257d..c0054e3515df 100644
--- a/translations/de-DE/content/github/setting-up-and-managing-organizations-and-teams/restricting-repository-visibility-changes-in-your-organization.md
+++ b/translations/de-DE/content/github/setting-up-and-managing-organizations-and-teams/restricting-repository-visibility-changes-in-your-organization.md
@@ -9,7 +9,7 @@ versions:
github-ae: '*'
---
-Du kannst die Möglichkeit der Änderung der Sichtbarkeit von Repositorys ausschließlich auf Organisationsinhaber beschränken oder auch Mitgliedern mit Administratorberechtigungen für ein Repository erlauben, die Sichtbarkeit des Repositorys von privat auf öffentlich oder von öffentlich auf privat zu ändern.
+You can restrict the ability to change repository visibility to organization owners only, or allow members with admin privileges for a repository to also change visibility.
{% warning %}
@@ -24,3 +24,7 @@ Du kannst die Möglichkeit der Änderung der Sichtbarkeit von Repositorys aussch
{% data reusables.organizations.member-privileges %}
5. Deaktiviere unter „Repository visibility change“ (Änderung der Sichtbarkeit von Repositorys) das Kontrollkästchen **Allow members to change repository visibilities for this organization** (Mitgliedern die Änderung der Sichtbarkeit von Repositorys für diese Organisation erlauben). 
6. Klicke auf **Save** (Speichern).
+
+### Weiterführende Informationen
+
+- „[Über Sichtbarkeit von Repositorys](/github/creating-cloning-and-archiving-repositories/about-repository-visibility)"
diff --git a/translations/de-DE/content/github/setting-up-and-managing-organizations-and-teams/reviewing-the-audit-log-for-your-organization.md b/translations/de-DE/content/github/setting-up-and-managing-organizations-and-teams/reviewing-the-audit-log-for-your-organization.md
index 12d028716278..1c3a69af505d 100644
--- a/translations/de-DE/content/github/setting-up-and-managing-organizations-and-teams/reviewing-the-audit-log-for-your-organization.md
+++ b/translations/de-DE/content/github/setting-up-and-managing-organizations-and-teams/reviewing-the-audit-log-for-your-organization.md
@@ -397,10 +397,10 @@ For more information, see "[Restricting publication of {% data variables.product
| Action | Description
|------------------|-------------------
-| `access` | Triggered when a repository owned by an organization is [switched from "private" to "public"](/articles/making-a-private-repository-public) (or vice versa).
+| `access` | Triggered when a user [changes the visibility](/github/administering-a-repository/setting-repository-visibility) of a repository in the organization.
| `add_member` | Triggered when a user accepts an [invitation to have collaboration access to a repository](/articles/inviting-collaborators-to-a-personal-repository).
| `add_topic` | Triggered when a repository admin [adds a topic](/articles/classifying-your-repository-with-topics) to a repository.
-| `archived` | Triggered when a repository admin [archives a repository](/articles/about-archiving-repositories).{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
+| `archived` | Triggered when a repository admin [archives a repository](/articles/about-archiving-repositories).{% if enterpriseServerVersions contains currentVersion %}
| `config.disable_anonymous_git_access` | Triggered when [anonymous Git read access is disabled](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository) in a public repository.
| `config.enable_anonymous_git_access` | Triggered when [anonymous Git read access is enabled](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository) in a public repository.
| `config.lock_anonymous_git_access` | Triggered when a repository's [anonymous Git read access setting is locked](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access).
@@ -428,9 +428,9 @@ For more information, see "[Restricting publication of {% data variables.product
| Action | Description
|------------------|-------------------
| `close` | Triggered when someone closes a security advisory. For more information, see "[About {% data variables.product.prodname_dotcom %} Security Advisories](/github/managing-security-vulnerabilities/about-github-security-advisories)."
-| `cve_request` | Triggered when someone requests a CVE (Common Vulnerabilities and Exposures) number from {% data.variables.product.prodname_dotcom %} for a draft security advisory.
-| `github_broadcast` | Triggered when {% data.variables.product.prodname_dotcom %} makes a security advisory public in the {% data variables.product.prodname_advisory_database %}.
-| `github_withdraw` | Triggered when {% data.variables.product.prodname_dotcom %} withdraws a security advisory that was published in error.
+| `cve_request` | Triggered when someone requests a CVE (Common Vulnerabilities and Exposures) number from {% data variables.product.prodname_dotcom %} for a draft security advisory.
+| `github_broadcast` | Triggered when {% data variables.product.prodname_dotcom %} makes a security advisory public in the {% data variables.product.prodname_advisory_database %}.
+| `github_withdraw` | Triggered when {% data variables.product.prodname_dotcom %} withdraws a security advisory that was published in error.
| `open` | Triggered when someone opens a draft security advisory.
| `publish` | Triggered when someone publishes a security advisory.
| `reopen` | Triggered when someone reopens as draft security advisory.
@@ -474,7 +474,7 @@ For more information, see "[Restricting publication of {% data variables.product
| Action | Description
|------------------|-------------------
-| `authorized_users_teams` | Triggered when an organization owner or a person with admin permissions to the repository updates the list of people or teams authorized to receive {% data variables.product.prodname_dependabot_alerts %} for vulnerable dependencies in the repository. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-dependabot-alerts)."
+| `authorized_users_teams` | Triggered when an organization owner or a person with admin permissions to the repository updates the list of people or teams authorized to receive {% data variables.product.prodname_dependabot_alerts %} for vulnerable dependencies in the repository. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-security-alerts)."
| `disable` | Triggered when a repository owner or person with admin access to the repository disables {% data variables.product.prodname_dependabot_alerts %}.
| `enable` | Triggered when a repository owner or person with admin access to the repository enables {% data variables.product.prodname_dependabot_alerts %}.
diff --git a/translations/de-DE/content/github/setting-up-and-managing-your-enterprise/about-enterprise-accounts.md b/translations/de-DE/content/github/setting-up-and-managing-your-enterprise/about-enterprise-accounts.md
index 79bd54753605..bc675d99275b 100644
--- a/translations/de-DE/content/github/setting-up-and-managing-your-enterprise/about-enterprise-accounts.md
+++ b/translations/de-DE/content/github/setting-up-and-managing-your-enterprise/about-enterprise-accounts.md
@@ -20,7 +20,7 @@ Mit einem Unternehmens-Konto kannst Du mehrere {% data variables.product.prodnam
- Sicherheit (Single-Sign-On, Zwei-Faktor-Authentifizierung)
- Anfragen und Unterstützen von Bundle-Sharing mit {% data variables.contact.enterprise_support %}
-{% data reusables.enterprise-accounts.enterprise-accounts-billing %}
+{% data reusables.enterprise-accounts.enterprise-accounts-billing %} For more information about managing your {% data variables.product.prodname_ghe_cloud %} subscription, see "[Viewing the subscription and usage for your enterprise account](/articles/viewing-the-subscription-and-usage-for-your-enterprise-account)." For more information about managing your {% data variables.product.prodname_ghe_server %} billing settings, see "[Managing billing for your enterprise](/admin/overview/managing-billing-for-your-enterprise)."
Weitere Informationen über die Unterschiede zwischen {% data variables.product.prodname_ghe_cloud %} und {% data variables.product.prodname_ghe_server %} findest Du auf „[Produkte von {% data variables.product.prodname_dotcom %}](/articles/githubs-products)." Um auf {% data variables.product.prodname_enterprise %} zu hochzustufen oder um mit einem Unternehmenskonto einzusteigen, kontaktiere bitte {% data variables.contact.contact_enterprise_sales %}.
diff --git a/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/about-your-profile.md b/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/about-your-profile.md
index 6fc4e02f5057..03bda1779647 100644
--- a/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/about-your-profile.md
+++ b/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/about-your-profile.md
@@ -13,7 +13,7 @@ versions:
Du kannst persönliche Informationen über Dich selbst in Deiner Bio hinzufügen, beispielsweise über frühere Orte, an denen Du gearbeitet hast, Projekte, an denen Du mitgewirkt hast, oder Interessen, die Du hast, von denen andere Personen möglicherweise wissen möchten. Weitere Informationen findest Du unter „[Bio zu Deinem Profil hinzufügen](/articles/personalizing-your-profile/#adding-a-bio-to-your-profile).“
-{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
{% data reusables.profile.profile-readme %}
@@ -23,19 +23,17 @@ Du kannst persönliche Informationen über Dich selbst in Deiner Bio hinzufügen
Personen, die Dein Profil aufrufen, sehen eine Zeitleiste Deiner Beitragsaktivitäten, wie Issues und Pull Requests, die Du geöffnet hast, Commits, die Du durchgeführt hast, und Pull Requests, deren Review Du durchgeführt hast. Du kannst wählen, ob Du nur öffentliche Beiträge anzeigen oder auch private, anonymisierte Beiträge einbeziehen möchtest. Weitere Informationen findest Du unter „[Beiträge auf Deiner Profilseite anzeigen](/articles/viewing-contributions-on-your-profile-page)“ oder „[Private Beiträge in Deinem Profil veröffentlichen oder verbergen](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile).“
-Außerdem ist Folgendes sichtbar:
+People who visit your profile can also see the following information.
-- Repositories and gists you own or contribute to. You can showcase your best work by pinning repositories and gists to your profile. Weitere Informationen finden Sie unter „[Elemente an Ihr Profil anheften](/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile)“.
-- Repositorys, die Sie mit einem Stern versehen haben. Weitere Informationen finden Sie unter „[Repositorys mit Sternen speichern](/articles/saving-repositories-with-stars/)“.
-- Eine Übersicht über Deine Aktivitäten in den Organisationen, Repositorys und Teams, in denen Du am meisten aktiv bist. For more information, see "[Showing an overview of your activity on your profile](/articles/showing-an-overview-of-your-activity-on-your-profile).{% if currentVersion == "free-pro-team@latest" %}
-- Badges that advertise your participation in programs like the {% data variables.product.prodname_arctic_vault %}, {% data variables.product.prodname_sponsors %}, or the {% data variables.product.company_short %} Developer Program.
-- Bei Verwendung von {% data variables.product.prodname_pro %}. Weitere Informationen findest Du unter „[Dein Profil personalisieren](/articles/personalizing-your-profile)“.{% endif %}
+- Repositories and gists you own or contribute to. {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}You can showcase your best work by pinning repositories and gists to your profile. For more information, see "[Pinning items to your profile](/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile)."{% endif %}
+- Repositorys, die Sie mit einem Stern versehen haben. For more information, see "[Saving repositories with stars](/articles/saving-repositories-with-stars/)."
+- Eine Übersicht über Deine Aktivitäten in den Organisationen, Repositorys und Teams, in denen Du am meisten aktiv bist. For more information, see "[Showing an overview of your activity on your profile](/articles/showing-an-overview-of-your-activity-on-your-profile)."{% if currentVersion == "free-pro-team@latest" %}
+- Badges that show if you use {% data variables.product.prodname_pro %} or participate in programs like the {% data variables.product.prodname_arctic_vault %}, {% data variables.product.prodname_sponsors %}, or the {% data variables.product.company_short %} Developer Program. Weitere Informationen findest Du unter „[Dein Profil personalisieren](/github/setting-up-and-managing-your-github-profile/personalizing-your-profile#displaying-badges-on-your-profile)“.{% endif %}
Sie können auch einen Status auf Ihrem Profil einstellen, um Angaben zu Ihrer Verfügbarkeit zu machen. Weitere Informationen findest Du unter „[Status festlegen](/articles/personalizing-your-profile/#setting-a-status).“
### Weiterführende Informationen
- „[Wie lege ich mein Profilbild fest?](/articles/how-do-i-set-up-my-profile-picture)“
-- „[Repositorys an Dein Profil anheften](/articles/pinning-repositories-to-your-profile)“
- „[Private Beiträge in Deinem Profil veröffentlichen oder verbergen](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)“
- „"[Beiträge auf Deinem Profil anzeigen](/articles/viewing-contributions-on-your-profile)“
diff --git a/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme.md b/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme.md
index e0e6ebe0bd03..023714da6b5b 100644
--- a/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme.md
+++ b/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme.md
@@ -4,7 +4,6 @@ intro: 'You can add a README to your {% data variables.product.prodname_dotcom %
versions:
free-pro-team: '*'
enterprise-server: '>=2.22'
- github-ae: '*'
---
### About your profile README
diff --git a/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/personalizing-your-profile.md b/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/personalizing-your-profile.md
index 9d12bf9da99e..272c5695167c 100644
--- a/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/personalizing-your-profile.md
+++ b/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/personalizing-your-profile.md
@@ -50,9 +50,9 @@ Du kannst den Namen, der in Deinem Profil angezeigt wird, ändern. This name may
Fügen Sie eine Biografie zu Ihrem Profil hinzu, um anderen {% data variables.product.product_name %}-Benutzern Informationen zu Ihrer Person bereitzustellen. Mit [@Erwähnungen](/articles/basic-writing-and-formatting-syntax) und Emojis kannst Du Informationen dazu angeben, wo Du gerade arbeitest oder früher gearbeitet hast, welche Tätigkeit Du ausübst oder welche Kaffeesorte Du trinkst.
-{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
-For a longer-form and more prominent way of displaying customized information about yourself, you can also use a profile README. For more information on the profile README, see "[Managing your profile README](/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme)."
+For a longer-form and more prominent way of displaying customized information about yourself, you can also use a profile README. For more information, see "[Managing your profile README](/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme)."
{% endif %}
diff --git a/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile.md b/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile.md
index 7cee1f5583dd..dd78bdf45cde 100644
--- a/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile.md
+++ b/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile.md
@@ -7,7 +7,6 @@ redirect_from:
versions:
free-pro-team: '*'
enterprise-server: '*'
- github-ae: '*'
---
Du kannst ein öffentliches Repository anheften, wenn Dir das Repository gehört oder wenn Du Beiträge zu dem Repository geleistet hast. Commits zu Forks zählen nicht als Beiträge. Daher kannst Du Forks, die Du nicht besitzt, auch nicht anheften. Weitere Informationen findest Du unter „[Warum werden meine Beiträge nicht in meinem Profil angezeigt?](/articles/why-are-my-contributions-not-showing-up-on-my-profile)“
diff --git a/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/publicizing-or-hiding-your-private-contributions-on-your-profile.md b/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/publicizing-or-hiding-your-private-contributions-on-your-profile.md
index 8bced3324eff..669a4402d3f0 100644
--- a/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/publicizing-or-hiding-your-private-contributions-on-your-profile.md
+++ b/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/publicizing-or-hiding-your-private-contributions-on-your-profile.md
@@ -1,6 +1,6 @@
---
title: Private Beiträge in Deinem Profil veröffentlichen oder verbergen
-intro: 'Ihr {% data variables.product.product_name %}-Profil zeigt ein Diagramm Ihrer Repository-Beiträge des letzten Jahres an. Du kannst auswählen, ob neben den Aktivitäten in öffentlichen Repositorys auch anonymisierte Aktivitäten in privaten Repositorys angezeigt werden sollen.'
+intro: 'Ihr {% data variables.product.product_name %}-Profil zeigt ein Diagramm Ihrer Repository-Beiträge des letzten Jahres an. You can choose to show anonymized activity from {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" %}private and internal{% else %}private{% endif %} repositories{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %} in addition to the activity from public repositories{% endif %}.'
redirect_from:
- /articles/publicizing-or-hiding-your-private-contributions-on-your-profile
versions:
diff --git a/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/viewing-contributions-on-your-profile.md b/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/viewing-contributions-on-your-profile.md
index dba161a04f57..0cf94c216be3 100644
--- a/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/viewing-contributions-on-your-profile.md
+++ b/translations/de-DE/content/github/setting-up-and-managing-your-github-profile/viewing-contributions-on-your-profile.md
@@ -1,6 +1,6 @@
---
title: Beiträge auf Deinem Profil anzeigen
-intro: 'Ihr {% data variables.product.product_name %}-Profil zeigt Ihre angehefteten Repositorys und ein Diagramm mit Ihren Repository-Beiträgen des letzten Jahres an.'
+intro: 'Your {% data variables.product.product_name %} profile shows off {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}your pinned repositories as well as{% endif %} a graph of your repository contributions over the past year.'
redirect_from:
- /articles/viewing-contributions/
- /articles/viewing-contributions-on-your-profile-page/
@@ -11,7 +11,7 @@ versions:
github-ae: '*'
---
-Dein Beteiligungsdiagramm zeigt die Aktivitäten in öffentlichen Repositorys. Du kannst die Aktivitäten in öffentlichen und privaten Repositorys anzeigen, wobei spezifische Details Deiner Aktivität in privaten Repositorys anonymisiert werden. Weitere Informationen finden Sie unter „[Private Beiträge in Ihrem Profil veröffentlichen oder verbergen](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)“.
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}Your contribution graph shows activity from public repositories. {% endif %}You can choose to show activity from {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}both public and{% endif %}private repositories, with specific details of your activity in private repositories anonymized. Weitere Informationen finden Sie unter „[Private Beiträge in Ihrem Profil veröffentlichen oder verbergen](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)“.
{% note %}
@@ -33,16 +33,20 @@ Bestimmte Aktionen zählen auf Deiner Profilseite als Beiträge:
### Beliebte Repositorys
-Dieser Abschnitt zeigt Deine Repositorys mit den meisten Beobachtern (Watcher) an. Wenn Du [Repositorys an Dein Profil anheftest](/articles/pinning-repositories-to-your-profile), wird dieser Abschnitt in „Pinned repositories“ (Angeheftete Repositorys) geändert.
+Dieser Abschnitt zeigt Deine Repositorys mit den meisten Beobachtern (Watcher) an. {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}Once you [pin repositories to your profile](/articles/pinning-repositories-to-your-profile), this section will change to "Pinned repositories."{% endif %}

+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
### Angeheftete Repositorys
Dieser Abschnitt zeigt bis zu sechs öffentliche Repositorys an und kann sowohl Deine Repositorys als auch die Repositorys enthalten, an denen Du Dich beteiligt hast. Damit Du ohne Weiteres wichtige Details zu den ausgewählten Repositorys anzeigen kannst, enthält jedes Repository in diesem Abschnitt eine Zusammenfassung der zu erledigenden Arbeit, die Anzahl der [Sterne](/articles/saving-repositories-with-stars/) des Repositorys und die im Repository verwendete Hauptprogrammiersprache. Weitere Informationen findest Du unter „[Repositorys an Dein Profil anheften](/articles/pinning-repositories-to-your-profile).“

+{% endif %}
+
### Beitragskalender
Dein Beitragskalender zeigt Deine Beitragsaktivität an.
@@ -72,11 +76,11 @@ Zeitstempel werden für Commits und Pull Requests unterschiedlich berechnet:

-Die in der Aktivitätsübersicht angezeigten Organisationen werden dementsprechend priorisiert, wie aktiv Du in der Organisation bist. Wenn Du in Deiner Profil-Bio eine Organisation @erwähnst und Du ein Organisationsmitglied bist, wird diese Organisation in der Aktivitätsübersicht priorisiert. Weitere Informationen findest Du unter „[Personen und Teams erwähnen](/articles/basic-writing-and-formatting-syntax/#mentioning-people-and-teams)“ oder „[Eine Biografie zu Deinem Profil hinzufügen](/articles/adding-a-bio-to-your-profile/).“
+Die in der Aktivitätsübersicht angezeigten Organisationen werden dementsprechend priorisiert, wie aktiv Sie in der Organisation sind. Wenn Sie in Ihrer Profil-Bio eine Organisation @erwähnen und Sie ein Organisationsmitglied sind, wird diese Organisation in der Aktivitätsübersicht priorisiert. Weitere Informationen finden Sie unter „[Personen und Teams erwähnen](/articles/basic-writing-and-formatting-syntax/#mentioning-people-and-teams)“ oder „[Eine Biografie zu Ihrem Profil hinzufügen](/articles/adding-a-bio-to-your-profile/)“.
### Beitragsaktivität
-Der Abschnitt für die Beitragsaktivität enthält eine detaillierte Zeitleiste zu Deiner Arbeit. Dazu zählen die von Dir erstellten oder mitverfassten Commits, die von Dir vorgeschlagenen Pull Requests und die von Dir geöffneten Issues. Du kannst Deine über einen gewissen Zeitraum erstellten Beiträge anzeigen. Klicke dazu im unteren Bereich Deiner Beitragsaktivität auf **Show more activity** (Mehr Aktivität anzeigen) oder im rechten Bereich der Seite auf das gewünschte Jahr. Wichtige Momente werden in Deiner Beitragsaktivität hervorgehoben. Dazu zählen Dein Beitrittsdatum zu einer Organisation, Dein erster vorgeschlagener Pull Request oder das Öffnen eines hochrangigen Issues. Wenn Du bestimmte Ereignisse auf Deiner Zeitleiste nicht siehst, solltest Du sicherstellen, dass Du weiterhin auf die Organisation oder das Repository zugreifen kannst, in der respektive in dem das Ereignis auftrat.
+Der Abschnitt für die Beitragsaktivität enthält eine detaillierte Zeitleiste zu Ihrer Arbeit. Dazu zählen die von Ihnen erstellten oder mitverfassten Commits, die von Ihnen vorgeschlagenen Pull Requests und die von Ihnen geöffneten Issues. Du kannst Deine über einen gewissen Zeitraum erstellten Beiträge anzeigen. Klicke dazu im unteren Bereich Deiner Beitragsaktivität auf **Show more activity** (Mehr Aktivität anzeigen) oder im rechten Bereich der Seite auf das gewünschte Jahr. Wichtige Momente werden in Ihrer Beitragsaktivität hervorgehoben. Dazu zählen Ihr Beitrittsdatum zu einer Organisation, Ihr erster vorgeschlagener Pull Request oder das Öffnen eines hochrangigen Issues. Wenn Sie bestimmte Ereignisse auf Ihrer Zeitleiste nicht anzeigen können, sollten Sie sicherstellen, dass Sie weiterhin auf die Organisation oder das Repository zugreifen können, in der bzw. in dem das Ereignis auftrat.

diff --git a/translations/de-DE/content/github/setting-up-and-managing-your-github-user-account/about-your-personal-dashboard.md b/translations/de-DE/content/github/setting-up-and-managing-your-github-user-account/about-your-personal-dashboard.md
index bfea95795bbb..e7fe7874c96d 100644
--- a/translations/de-DE/content/github/setting-up-and-managing-your-github-user-account/about-your-personal-dashboard.md
+++ b/translations/de-DE/content/github/setting-up-and-managing-your-github-user-account/about-your-personal-dashboard.md
@@ -15,7 +15,7 @@ versions:
Ihr persönliches Dashboard ist die erste Seite, die Sie sehen, wenn Sie sich bei {% data variables.product.product_name %} anmelden.
-Um nach der Anmeldung auf Ihr persönliches Dashboard zuzugreifen, klicken Sie auf das {% octicon "mark-github" aria-label="The github octocat logo" %} in der oberen linken Ecke einer beliebigen Seite auf {% data variables.product.product_url %}.
+Um nach der Anmeldung auf Ihr persönliches Dashboard zuzugreifen, klicken Sie auf das {% octicon "mark-github" aria-label="The github octocat logo" %} in der oberen linken Ecke einer beliebigen Seite auf {% data variables.product.product_name %}.
### Neueste Aktivitäten finden
@@ -39,11 +39,11 @@ Im Abschnitt „All activity" (Alle Aktivitäten) in Deinem Newsfeed kannst Du A
In Ihrem News-Feed werden Aktualisierungen angezeigt, wenn ein Benutzer, dem Sie folgen,
- Ein Repository mit einem Stern versieht.
-- Einem anderen Benutzer folgt.
-- ein öffentliches Repository erstellt.
+- Follows another user.{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- Creates a public repository.{% endif %}
- Einen Issue oder Pull Request mit der Kennzeichnung „help wanted“ oder „good first issue“ in einem von Dir beobachteten Repository öffnet.
-- Commits an ein von Dir beobachtetes Repository freigibt.
-- Ein öffentliches Repository forkt.
+- Pushes commits to a repository you watch.{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- Forks a public repository.{% endif %}
Weitere Informationen zu Sternen für Repositorys und zum Folgen von Personen finden Sie unter „[Repositorys mit Sternen speichern ](/articles/saving-repositories-with-stars/)“ und „[Jemandem folgen](/articles/following-people)“.
diff --git a/translations/de-DE/content/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository.md b/translations/de-DE/content/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository.md
index 524986a73ef0..dfbe9bbdd68e 100644
--- a/translations/de-DE/content/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository.md
+++ b/translations/de-DE/content/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository.md
@@ -50,7 +50,7 @@ Repositorys, die einer Organisation gehören, können feiner abgestufte Zugriffs
### Weiterführende Informationen
-- „[Berechtigungsebenen für ein Repository eines Benutzerkontos](/articles/permission-levels-for-a-user-account-repository/#collaborator-access-on-a-repository-owned-by-a-user-account)“
+- „[Berechtigungsebenen für ein Repository eines Benutzerkontos](/articles/permission-levels-for-a-user-account-repository/#collaborator-access-for-a-repository-owned-by-a-user-account)“
- „[Mitarbeiter aus einem persönlichen Repository entfernen](/articles/removing-a-collaborator-from-a-personal-repository)“
- „[Dich selbst aus dem Repository eines Mitarbeiters entfernen](/articles/removing-yourself-from-a-collaborator-s-repository)“
- „[Mitglieder in Teams organisieren](/articles/organizing-members-into-teams)“
diff --git a/translations/de-DE/content/github/setting-up-and-managing-your-github-user-account/permission-levels-for-a-user-account-repository.md b/translations/de-DE/content/github/setting-up-and-managing-your-github-user-account/permission-levels-for-a-user-account-repository.md
index f157c744a115..8a12a261db2f 100644
--- a/translations/de-DE/content/github/setting-up-and-managing-your-github-user-account/permission-levels-for-a-user-account-repository.md
+++ b/translations/de-DE/content/github/setting-up-and-managing-your-github-user-account/permission-levels-for-a-user-account-repository.md
@@ -1,6 +1,6 @@
---
title: Berechtigungsebenen für ein Repository eines Benutzerkontos
-intro: 'Ein Repository, das einem Benutzerkonto gehört, hat zwei Berechtigungsebenen: den *Repository-Inhaber* und die *Mitarbeiter*.'
+intro: 'A repository owned by a user account has two permission levels: the repository owner and collaborators.'
redirect_from:
- /articles/permission-levels-for-a-user-account-repository
versions:
@@ -9,38 +9,48 @@ versions:
github-ae: '*'
---
+### About permissions levels for a user account repository
+
+Repositories owned by user accounts have one owner. Ownership permissions can't be shared with another user account.
+
+You can also {% if currentVersion == "free-pro-team@latest" %}invite{% else %}add{% endif %} users on {% data variables.product.product_name %} to your repository as collaborators. For more information, see "[Inviting collaborators to a personal repository](/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository)."
+
{% tip %}
-**Tipp:** Wenn Du einen feiner abgestuften Lese-/Schreibzugriff auf ein Repository benötigst, das Deinem Benutzerkonto gehört, kannst Du das Repository an eine Organisation übertragen. Weitere Informationen findest Du unter „[Ein Repository übertragen](/articles/transferring-a-repository).“
+**Tip:** If you require more granular access to a repository owned by your user account, consider transferring the repository to an organization. Weitere Informationen finden Sie unter „[Ein Repository übertragen](/github/administering-a-repository/transferring-a-repository#transferring-a-repository-owned-by-your-user-account)“.
{% endtip %}
-#### Inhaberzugriff auf ein Repository eines Benutzerkontos
-
-Der Repository-Inhaber besitzt die vollständige Kontrolle über das Repository. Neben den Berechtigungen, die auch Repository-Mitarbeitern erteilt werden, stehen dem Repository-Inhaber zusätzlich folgende Möglichkeiten zur Verfügung:
-
-- {% if currentVersion == "free-pro-team@latest" %}[Invite collaborators](/articles/inviting-collaborators-to-a-personal-repository){% else %}[Add collaborators](/articles/inviting-collaborators-to-a-personal-repository){% endif %}
-- Change the visibility of the repository (from [public to private](/articles/making-a-public-repository-private), or from [private to public](/articles/making-a-private-repository-public)){% if currentVersion == "free-pro-team@latest" %}
-- [Interaktionen mit einem Repository einschränken](/articles/limiting-interactions-with-your-repository){% endif %}
-- Einen Pull Request auf einem geschützten Branch zusammenführen, selbst ohne genehmigende Reviews
-- [Das Repository löschen](/articles/deleting-a-repository)
-- [Manage a repository's topics](/articles/classifying-your-repository-with-topics){% if currentVersion == "free-pro-team@latest" %}
-- Manage security and analysis settings. For more information, see "[Managing security and analysis settings for your user account](/github/setting-up-and-managing-your-github-user-account/managing-security-and-analysis-settings-for-your-user-account)."{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-- [Enable the dependency graph](/github/visualizing-repository-data-with-graphs/exploring-the-dependencies-and-dependents-of-a-repository) for a private repository{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-- Pakete löschen. Weitere Informationen findest Du unter „[Ein Paket löschen](/github/managing-packages-with-github-packages/deleting-a-package)."{% endif %}
-- soziale Tickets für Repositorys erstellen und bearbeiten (siehe „[Social-Media-Vorschau Ihres Repositorys anpassen](/articles/customizing-your-repositorys-social-media-preview)“)
-- das Repository in eine Vorlage umwandeln For more information, see "[Creating a template repository](/articles/creating-a-template-repository)."{% if currentVersion != "github-ae@latest" %}
-- Receive [{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot_alerts %}{% else %}security alerts{% endif %} for vulnerable dependencies](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies) in a repository.{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-- Dismiss {% data variables.product.prodname_dependabot_alerts %} in your repository. For more information, see "[Viewing and updating vulnerable dependencies in your repository](/github/managing-security-vulnerabilities/viewing-and-updating-vulnerable-dependencies-in-your-repository)."
-- [Manage data usage for your private repository](/github/understanding-how-github-uses-and-protects-your-data/managing-data-use-settings-for-your-private-repository){% endif %}
-- [Codeinhaber für das Repository definieren](/articles/about-code-owners)
-- [Archive repositories](/articles/about-archiving-repositories){% if currentVersion == "free-pro-team@latest" %}
-- Sicherheitshinweise erstellen. Weitere Informationen findest Du unter „[ Über {% data variables.product.prodname_security_advisories %}](/github/managing-security-vulnerabilities/about-github-security-advisories)."
-- eine Sponsorenschaltfläche anzeigen Weitere Informationen findest Du unter „[Sponsorenschaltfläche in Deinem Repository anzeigen](/articles/displaying-a-sponsor-button-in-your-repository)“{% endif %}
-
-Bei einem Repository, das einem Benutzerkonto gehört, gibt es nur **einen Inhaber**. Diese Berechtigung kann nicht mit einem anderem Benutzerkonto geteilt werden. Informationen zur Übertragung der Repository-Inhaberschaft auf einen anderen Benutzer findest Du unter „[Ein Repository übertragen](/articles/how-to-transfer-a-repository).“
-
-#### Mitarbeiterzugriff auf ein Repository eines Benutzerkontos
+### Owner access for a repository owned by a user account
+
+Der Repository-Inhaber besitzt die vollständige Kontrolle über das Repository. In addition to the actions that any collaborator can perform, the repository owner can perform the following actions.
+
+| Aktion | Weitere Informationen |
+|:---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |:----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| {% if currentVersion == "free-pro-team@latest" %}Invite collaborators{% else %}Add collaborators{% endif %} | |
+| „[Mitarbeiter in ein persönliches Repository einladen](/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository)“ | |
+| Change the visibility of the repository | "[Setting repository visibility](/github/administering-a-repository/setting-repository-visibility)" |{% if currentVersion == "free-pro-team@latest" %}
+| Limit interactions with the repository | "[Limiting interactions in your repository](/github/building-a-strong-community/limiting-interactions-in-your-repository)" |{% endif %}
+| Einen Pull Request auf einem geschützten Branch zusammenführen, selbst ohne genehmigende Reviews | „[Informationen zu geschützten Branches](/github/administering-a-repository/about-protected-branches)“ |
+| Das Repository löschen | „[Repository löschen](/github/administering-a-repository/deleting-a-repository)“ |
+| Manage the repository's topics | "[Classifying your repository with topics](/github/administering-a-repository/classifying-your-repository-with-topics)" |{% if currentVersion == "free-pro-team@latest" %}
+| Manage security and analysis settings for the repository | "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository)" |{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| Enable the dependency graph for a private repository | "[Exploring the dependencies of a repository](/github/visualizing-repository-data-with-graphs/exploring-the-dependencies-of-a-repository#enabling-and-disabling-the-dependency-graph-for-a-private-repository)" |{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| Pakete löschen | "[Deleting a package](/github/managing-packages-with-github-packages/deleting-a-package)" |{% endif %}
+| Customize the repository's social media preview | "[Customizing your repository's social media preview](/github/administering-a-repository/customizing-your-repositorys-social-media-preview)" |
+| Create a template from the repository | "[Creating a template repository](/github/creating-cloning-and-archiving-repositories/creating-a-template-repository)" |{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+| Receive | |
+| {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot_alerts %}{% else %}security alerts{% endif %} for vulnerable dependencies | "[About alerts for vulnerable dependencies](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies)" |{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| Dismiss {% data variables.product.prodname_dependabot_alerts %} in the repository | „[Angreifbare Abhängigkeiten in Ihrem Repository anzeigen und aktualisieren](/github/managing-security-vulnerabilities/viewing-and-updating-vulnerable-dependencies-in-your-repository)“ |
+| Manage data use for a private repository | "[Managing data use settings for your private repository](/github/understanding-how-github-uses-and-protects-your-data/managing-data-use-settings-for-your-private-repository)"|{% endif %}
+| Codeinhaber für das Repository definieren | „[Informationen zu Codeinhabern](/github/creating-cloning-and-archiving-repositories/about-code-owners)“ |
+| Archive the repository | "[About archiving repositories](/github/creating-cloning-and-archiving-repositories/about-archiving-repositories)" |{% if currentVersion == "free-pro-team@latest" %}
+| Sicherheitshinweise erstellen | "[About {% data variables.product.prodname_security_advisories %}](/github/managing-security-vulnerabilities/about-github-security-advisories)" |
+| Eine Sponsorenschaltfläche anzeigen | "[Displaying a sponsor button in your repository](/github/administering-a-repository/displaying-a-sponsor-button-in-your-repository)" |{% endif %}
+
+### Collaborator access for a repository owned by a user account
+
+Collaborators on a personal repository can pull (read) the contents of the repository and push (write) changes to the repository.
{% note %}
@@ -48,27 +58,26 @@ Bei einem Repository, das einem Benutzerkonto gehört, gibt es nur **einen Inhab
{% endnote %}
-Mitarbeiter haben folgende Möglichkeiten in persönlichen Repositorys:
-
-- Etwas zum Repository pushen (schreiben), etwas vom Repository abrufen (lesen) und das Repository forken (kopieren)
-- Kennzeichnungen und Meilensteine erstellen, anwenden und löschen
-- Issues öffnen, schließen, erneut öffnen und zuweisen
-- Kommentare zu Commits, Pull Requests und Issues bearbeiten und löschen
-- Issues und Pull Requests als Duplikate markieren Weitere Informationen findest Du unter „[Informationen zu Duplikaten von Issues und Pull Requests](/articles/about-duplicate-issues-and-pull-requests)“
-- Pull Requests öffnen, zusammenführen und schließen
-- Vorgeschlagene Änderungen auf Pull Requests anwenden. Weitere Informationen findest Du unter „[Feedback in Deinen Pull Request aufnehmen](/articles/incorporating-feedback-in-your-pull-request).“
-- Send pull requests from forks of the repository{% if currentVersion == "free-pro-team@latest" %}
-- Pakete veröffentlichen, ansehen und installieren. Weitere Informationen findest Du unter „[Pakete veröffentlichen und verwalten](/github/managing-packages-with-github-packages/publishing-and-managing-packages)."{% endif %}
-- Wikis erstellen und bearbeiten
-- Erstellen und Bearbeiten von Releases. Weitere Informationen findest Du unter „[Releases in einem Repository verwalten](/github/administering-a-repository/managing-releases-in-a-repository).
-- Sich selbst als Mitarbeiter aus dem Repository entfernen
-- Einen Review zu einem Pull Request absenden, der seine Merge-Fähigkeit beeinflusst
-- Als designierter Codeinhaber des Repositorys agieren. Weitere Informationen findest Du unter „[Informationen zu Codeinhabern](/articles/about-code-owners).“
-- Eine Unterhaltung sperren. For more information, see "[Locking conversations](/articles/locking-conversations)."{% if currentVersion == "free-pro-team@latest" %}
-- missbräuchliche Inhalte an den {% data variables.contact.contact_support %} melden Weitere Informationen findest Du unter „[Missbrauch oder Spam melden](/articles/reporting-abuse-or-spam)“.{% endif %}
-- einen Issue in ein anderes Repository übertragen Weitere Informationen finden Sie unter „[Einen Issue in ein anderes Repository übertragen](/articles/transferring-an-issue-to-another-repository)“.
+Collaborators can also perform the following actions.
+
+| Aktion | Weitere Informationen |
+|:----------------------------------------------------------------------------------------- |:--------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| Fork the repository | „[Über Forks](/github/collaborating-with-issues-and-pull-requests/about-forks)" |
+| Create, edit, and delete comments on commits, pull requests, and issues in the repository | - "[About issues](/github/managing-your-work-on-github/about-issues)"
- "[Commenting on a pull request](/github/collaborating-with-issues-and-pull-requests/commenting-on-a-pull-request)"
- "[Managing disruptive comments](/github/building-a-strong-community/managing-disruptive-comments)"
|
+| Create, assign, close, and re-open issues in the repository | "[Managing your work with issues](/github/managing-your-work-on-github/managing-your-work-with-issues)" |
+| Manage labels for issues and pull requests in the repository | "[Labeling issues and pull requests](/github/managing-your-work-on-github/labeling-issues-and-pull-requests)" |
+| Manage milestones for issues and pull requests in the repository | „[Meilensteine für Issues und Pull Requests erstellen und bearbeiten](/github/managing-your-work-on-github/creating-and-editing-milestones-for-issues-and-pull-requests)“ |
+| Mark an issue or pull request in the repository as a duplicate | "[About duplicate issues and pull requests](/github/managing-your-work-on-github/about-duplicate-issues-and-pull-requests)" |
+| Create, merge, and close pull requests in the repository | "[Proposing changes to your work with pull requests](/github/collaborating-with-issues-and-pull-requests/proposing-changes-to-your-work-with-pull-requests)" |
+| Apply suggested changes to pull requests in the repository | "[Incorporating feedback in your pull request](/github/collaborating-with-issues-and-pull-requests/incorporating-feedback-in-your-pull-request)" |
+| Create a pull request from a fork of the repository | „[Einen Pull Request von einem Fork erstellen](/github/collaborating-with-issues-and-pull-requests/creating-a-pull-request-from-a-fork)“ |
+| Submit a review on a pull request that affects the mergeability of the pull request | „[Vorgeschlagene Änderungen in einem Pull Request überprüfen](/github/collaborating-with-issues-and-pull-requests/reviewing-proposed-changes-in-a-pull-request)" |
+| Create and edit a wiki for the repository | „[Informationen zu Wikis](/github/building-a-strong-community/about-wikis)“ |
+| Create and edit releases for the repository | "[Managing releases in a repository](/github/administering-a-repository/managing-releases-in-a-repository)" |
+| Act as a code owner for the repository | "[About code owners](/articles/about-code-owners)" |{% if currentVersion == "free-pro-team@latest" %}
+| Publish, view, or install packages | "[Publishing and managing packages](/github/managing-packages-with-github-packages/publishing-and-managing-packages)" |{% endif %}
+| sich selbst als Mitarbeiter aus dem Repository entfernen | „[Dich selbst aus dem Repository eines Mitarbeiters entfernen](/github/setting-up-and-managing-your-github-user-account/removing-yourself-from-a-collaborators-repository)“ |
### Weiterführende Informationen
-- „[Mitarbeiter in ein persönliches Repository einladen](/articles/inviting-collaborators-to-a-personal-repository)“
- „[Berechtigungsebenen für die Repositorys einer Organisation](/articles/repository-permission-levels-for-an-organization)“
diff --git a/translations/de-DE/content/github/site-policy/github-marketplace-terms-of-service.md b/translations/de-DE/content/github/site-policy/github-marketplace-terms-of-service.md
index 857e5ac03da6..68c1424375f7 100644
--- a/translations/de-DE/content/github/site-policy/github-marketplace-terms-of-service.md
+++ b/translations/de-DE/content/github/site-policy/github-marketplace-terms-of-service.md
@@ -6,11 +6,11 @@ versions:
free-pro-team: '*'
---
-Willkommen bei GitHub Marketplace ("Marketplace")! Wir freuen uns, dass Sie hier sind. Bitte lesen Sie diese Nutzungsbedingungen ("Marketplace-Bedingungen") sorgfältig durch, bevor Sie auf GitHub Marketplace zugreifen oder verwenden. GitHub Marketplace ist eine Plattform, mit der Sie Entwicklerprodukte (kostenlos oder gegen Gebühr) erwerben können, die mit Ihrem GitHub.com-Konto verwendet werden können ("Entwicklerprodukte"). Obwohl von GitHub, Inc. ("GitHub", "wir", "uns") verkauft, können Entwicklerprodukte entweder von GitHub oder von externen Softwareanbietern entwickelt und gewartet werden und erfordern unter Umständen die Zustimmung zu gesonderten Nutzungsbedingungen. Ihre Nutzung bzw. Ihr Kauf von Entwicklerprodukten unterliegt diesen Marketplace-Bedingungen und den anfallenden Gebühren und kann darüber hinaus zusätzlichen Bedingungen unterliegen, die vom Drittlizenzgeber dieses Entwicklerprodukts (dem "Produktanbieter") bereitgestellt werden.
+Willkommen bei GitHub Marketplace ("Marketplace")! Wir freuen uns, dass Sie hier sind. Bitte lesen Sie diese Nutzungsbedingungen ("Marketplace-Bedingungen") sorgfältig durch, bevor Sie auf GitHub Marketplace zugreifen oder verwenden. GitHub Marketplace is a platform that allows you to select developer apps or actions (for free or for a charge) that can be used with your GitHub.com account ("Developer Products"). Although offered by GitHub, Inc. ("GitHub", "we", "us"), Developer Products may be developed and maintained by either GitHub or by third-party software providers. Your selection or use of Developer Products is subject to these Marketplace Terms and any applicable fees, and may require you to agree to additional terms as provided by the third party licensor of that Developer Product (the "Product Provider").
Durch die Nutzung von Marketplace erklären Sie sich damit einverstanden, an diese Marketplace-Bedingungen gebunden zu sein.
-Datum des Inkrafttretens: 11. Oktober 2017
+Effective Date: November 20, 2020
### A. Nutzungsbedingungen von GitHub.com
@@ -40,11 +40,11 @@ Wenn Sie Fragen oder Bedenken bezüglich Ihrer Abrechnung haben, wenden Sie sich
### E. Ihre Daten und die Datenschutzrichtlinie von GitHub
-**Datenschutz.** Wenn Sie ein Entwicklerprodukt kaufen oder abonnieren, muss GitHub bestimmte personenbezogene Daten (wie in der [GitHub-Datenschutzerklärung](/articles/github-privacy-statement/) definiert) mit dem Produktanbieter teilen, um Ihnen das Entwicklerprodukt zur Verfügung zu stellen, unabhängig von Ihren Datenschutzeinstellungen. Abhängig von den Anforderungen des von Ihnen gewählten Entwicklerprodukts teilt GitHub möglicherweise lediglich den Namen Ihres Benutzerkontos, Ihre ID und Ihre primäre E-Mail-Adresse oder aber auch den Zugriff auf die Inhalte in Ihren Repositorys, einschließlich der Möglichkeit, Ihre privaten Daten einzusehen und zu ändern. Sie können den Umfang der Berechtigungen, die das Entwicklerprodukt erfordert, einsehen und diese akzeptieren oder ablehnen, wenn Sie die Berechtigung über OAuth erteilen. In Übereinstimmung mit der [GitHub-Datenschutzerklärung](/articles/github-privacy-statement/) werden wir dem Produktanbieter nur die für den Zweck der Transaktion erforderlichen Mindestinformationen zur Verfügung stellen.
+**Privacy.** When you select or use a Developer Product, GitHub may share certain Personal Information (as defined in the [GitHub Privacy Statement](/articles/github-privacy-statement/)) with the Product Provider (if any such Personal Information is received from you) in order to provide you with the Developer Product, regardless of your privacy settings. Abhängig von den Anforderungen des von Ihnen gewählten Entwicklerprodukts teilt GitHub möglicherweise lediglich den Namen Ihres Benutzerkontos, Ihre ID und Ihre primäre E-Mail-Adresse oder aber auch den Zugriff auf die Inhalte in Ihren Repositorys, einschließlich der Möglichkeit, Ihre privaten Daten einzusehen und zu ändern. Sie können den Umfang der Berechtigungen, die das Entwicklerprodukt erfordert, einsehen und diese akzeptieren oder ablehnen, wenn Sie die Berechtigung über OAuth erteilen.
-Wenn Sie Ihre Entwicklerproduktdienste kündigen und den Zugriff über Ihre Kontoeinstellungen widerrufen, kann der Produktanbieter nicht mehr auf Ihr Konto zugreifen. Der Produktanbieter ist dafür verantwortlich, Ihre personenbezogenen Daten innerhalb des definierten Zeitraums aus seinen Systemen zu löschen. Wenden Sie sich an den Produktanbieter, um sicherzustellen, dass Ihr Konto ordnungsgemäß gekündigt wurde.
+If you cease using the Developer Product and revoke access through your account settings, the Product Provider will no longer be able to access your account. Der Produktanbieter ist dafür verantwortlich, Ihre personenbezogenen Daten innerhalb des definierten Zeitraums aus seinen Systemen zu löschen. Wenden Sie sich an den Produktanbieter, um sicherzustellen, dass Ihr Konto ordnungsgemäß gekündigt wurde.
-**Haftungsausschluss für Datensicherheit.** Wenn Sie ein Entwicklerprodukt kaufen oder abonnieren, liegt die Sicherheit des Entwicklerprodukts und die Verwahrung Ihrer Daten in der Verantwortung des Produktanbieters. Sie sind dafür verantwortlich, die Sicherheitsaspekte des Kaufs und der Verwendung des Entwicklerprodukts für Ihre eigenen Sicherheits-, Risiko- und Konformitätsüberlegungen zu verstehen.
+**Data Security and Privacy Disclaimer.** When you select or use a Developer Product, the security of the Developer Product and the custodianship of your data, including your Personal Information (if any), is the responsibility of the Product Provider. You are responsible for understanding the security and privacy considerations of the selection or use of the Developer Product for your own security and privacy risk and compliance considerations.
### F. Rechte an Entwicklerprodukten
diff --git a/translations/de-DE/content/github/using-git/setting-your-username-in-git.md b/translations/de-DE/content/github/using-git/setting-your-username-in-git.md
index 95378aee7c06..00bad9aff70a 100644
--- a/translations/de-DE/content/github/using-git/setting-your-username-in-git.md
+++ b/translations/de-DE/content/github/using-git/setting-your-username-in-git.md
@@ -20,13 +20,13 @@ Eine Änderung Deines Namens für Git-Commits mit dem Befehl `git config` wirkt
2. {% data reusables.user_settings.set_your_git_username %}
```shell
$ git config --global user.name "Mona Lisa"
- ```
+ ```
3. {% data reusables.user_settings.confirm_git_username_correct %}
```shell
$ git config --global user.name
> Mona Lisa
- ```
+ ```
### Git-Benutzername für ein einzelnes Repository festlegen
@@ -37,13 +37,13 @@ Eine Änderung Deines Namens für Git-Commits mit dem Befehl `git config` wirkt
3. {% data reusables.user_settings.set_your_git_username %}
```shell
$ git config user.name "Mona Lisa"
- ```
+ ```
3. {% data reusables.user_settings.confirm_git_username_correct %}
```shell
$ git config user.name
> Mona Lisa
- ```
+ ```
### Weiterführende Informationen
diff --git a/translations/de-DE/content/github/using-git/which-remote-url-should-i-use.md b/translations/de-DE/content/github/using-git/which-remote-url-should-i-use.md
index 0181992503f1..fb5eff82e8e9 100644
--- a/translations/de-DE/content/github/using-git/which-remote-url-should-i-use.md
+++ b/translations/de-DE/content/github/using-git/which-remote-url-should-i-use.md
@@ -3,7 +3,7 @@ title: Welche Remote-URL sollte ich verwenden?
redirect_from:
- /articles/which-url-should-i-use/
- /articles/which-remote-url-should-i-use
-intro: 'Es gibt mehrere Möglichkeiten, Repositorys zu klonen, die auf {% data variables.product.prodname_dotcom %} vorhanden sind.'
+intro: 'Es gibt mehrere Möglichkeiten, Repositorys zu klonen, die auf {% data variables.product.product_location %} vorhanden sind.'
versions:
free-pro-team: '*'
enterprise-server: '*'
@@ -16,7 +16,7 @@ Informationen zum Festlegen oder Ändern Deiner Remote-URL findest Du unter „[
### Cloning with HTTPS URLs
-Die `https://`-Klon-URLs sind auf allen Repositorys, einschließlich der öffentlichen und privaten, verfügbar. Diese URLs funktionieren auch dann, wenn Du hinter einer Firewall oder einem Proxy stehst.
+The `https://` clone URLs are available on all repositories, regardless of visibility. `https://` clone URLs work even if you are behind a firewall or proxy.
Wenn Du an der Befehlszeile mittels HTTPS-URLs `git clone`-, `git fetch`-, `git pull`- oder `git push`-Befehle an ein Remote-Repository sendest, musst Du Deinen {% data variables.product.product_name %}-Benutzernamen und Dein Passwort eingeben. {% data reusables.user_settings.password-authentication-deprecation %}
diff --git a/translations/de-DE/content/github/writing-on-github/creating-gists.md b/translations/de-DE/content/github/writing-on-github/creating-gists.md
index d42f9c65c29b..58362dd3042f 100644
--- a/translations/de-DE/content/github/writing-on-github/creating-gists.md
+++ b/translations/de-DE/content/github/writing-on-github/creating-gists.md
@@ -33,7 +33,11 @@ In folgenden Fällen erhältst Du eine Benachrichtigung:
- Jemand erwähnt Dich in einem Gist.
- Du abonnierst einen Gist, indem Du oben im Gist auf **Subscribe** (Abonnieren) klickst.
-Du kannst Gists an Deinem Profil anheften, damit andere Personen sie leichter sehen. Weitere Informationen finden Sie unter „[Elemente an Ihr Profil anheften](/articles/pinning-items-to-your-profile)“.
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+Du kannst Gists an Deinem Profil anheften, damit andere Personen sie leichter sehen. Weitere Informationen findest Du unter „[Elemente an Dein Profil anheften](/articles/pinning-items-to-your-profile).“
+
+{% endif %}
Um Gists zu entdecken, die von anderen Benutzern erstellt wurden, rufe die {% data variables.gists.gist_homepage %} auf und klicke dort auf **All Gists** (Alle Gists). Daraufhin wird eine Seite mit allen Gists angezeigt, die nach dem Zeitpunkt der Erstellung oder Aktualisierung sortiert sind. Mit der {% data variables.gists.gist_search_url %} können Sie Gists auch nach Sprache suchen. Die Gist-Suche nutzt dieselbe Suchsyntax wie [die Codesuche](/articles/searching-code).
diff --git a/translations/de-DE/content/packages/managing-container-images-with-github-container-registry/deleting-a-container-image.md b/translations/de-DE/content/packages/managing-container-images-with-github-container-registry/deleting-a-container-image.md
index ddf6eae62764..3fd9ac99bd21 100644
--- a/translations/de-DE/content/packages/managing-container-images-with-github-container-registry/deleting-a-container-image.md
+++ b/translations/de-DE/content/packages/managing-container-images-with-github-container-registry/deleting-a-container-image.md
@@ -16,10 +16,6 @@ To delete a container image, you must have admin permissions to the container im
When deleting public packages, be aware that you may break projects that depend on your package.
-### Reserved package versions and names
-
-{% data reusables.package_registry.package-immutability %}
-
### Deleting a version of a user-owned container image on {% data variables.product.prodname_dotcom %}
{% data reusables.package_registry.package-settings-from-user-level %}
diff --git a/translations/de-DE/content/packages/publishing-and-managing-packages/deleting-a-package.md b/translations/de-DE/content/packages/publishing-and-managing-packages/deleting-a-package.md
index e1d44a2b9165..1826f0517a88 100644
--- a/translations/de-DE/content/packages/publishing-and-managing-packages/deleting-a-package.md
+++ b/translations/de-DE/content/packages/publishing-and-managing-packages/deleting-a-package.md
@@ -35,10 +35,6 @@ At this time, {% data variables.product.prodname_registry %} on {% data variable
{% endif %}
-### Reserved package versions and names
-
-{% data reusables.package_registry.package-immutability %}
-
### Deleting a version of a private package on {% data variables.product.product_name %}
To delete a private package version, you must have admin permissions in the repository.
diff --git a/translations/de-DE/content/packages/publishing-and-managing-packages/publishing-a-package.md b/translations/de-DE/content/packages/publishing-and-managing-packages/publishing-a-package.md
index 53b90403f19e..a5132243ebca 100644
--- a/translations/de-DE/content/packages/publishing-and-managing-packages/publishing-a-package.md
+++ b/translations/de-DE/content/packages/publishing-and-managing-packages/publishing-a-package.md
@@ -18,8 +18,6 @@ You can help people understand and use your package by providing a description a
{% data reusables.package_registry.public-or-private-packages %} A repository can contain more than one package. To prevent confusion, make sure the README and description clearly provide information about each package.
-{% data reusables.package_registry.package-immutability %}
-
{% if currentVersion == "free-pro-team@latest" %}
If a new version of a package fixes a security vulnerability, you should publish a security advisory in your repository.
{% data variables.product.prodname_dotcom %} reviews each published security advisory and may use it to send {% data variables.product.prodname_dependabot_alerts %} to affected repositories. For more information, see "[About GitHub Security Advisories](/github/managing-security-vulnerabilities/about-github-security-advisories)."
diff --git a/translations/de-DE/content/packages/using-github-packages-with-your-projects-ecosystem/configuring-npm-for-use-with-github-packages.md b/translations/de-DE/content/packages/using-github-packages-with-your-projects-ecosystem/configuring-npm-for-use-with-github-packages.md
index 271fc18da2ca..7e855a66023d 100644
--- a/translations/de-DE/content/packages/using-github-packages-with-your-projects-ecosystem/configuring-npm-for-use-with-github-packages.md
+++ b/translations/de-DE/content/packages/using-github-packages-with-your-projects-ecosystem/configuring-npm-for-use-with-github-packages.md
@@ -48,12 +48,15 @@ $ npm login --registry=https://npm.pkg.github.com
To authenticate by logging in to npm, use the `npm login` command, replacing *USERNAME* with your {% data variables.product.prodname_dotcom %} username, *TOKEN* with your personal access token, and *PUBLIC-EMAIL-ADDRESS* with your email address.
+If {% data variables.product.prodname_registry %} is not your default package registry for using npm and you want to use the `npm audit` command, we recommend you use the `--scope` flag with the owner of the package when you authenticate to {% data variables.product.prodname_registry %}.
+
{% if enterpriseServerVersions contains currentVersion %}
If your instance has subdomain isolation enabled:
{% endif %}
```shell
-$ npm login --registry=https://{% if currentVersion == "free-pro-team@latest" %}npm.pkg.github.com{% else %}npm.HOSTNAME/{% endif %}
+$ npm login --scope=@OWNER --registry=https://{% if currentVersion == "free-pro-team@latest" %}npm.pkg.github.com{% else %}npm.HOSTNAME/{% endif %}
+
> Username: USERNAME
> Password: TOKEN
> Email: PUBLIC-EMAIL-ADDRESS
@@ -63,9 +66,10 @@ $ npm login --registry=https://{% if currentVersion == "free-pro-team@latest" %}
If your instance has subdomain isolation disabled:
```shell
-registry=https://npm.pkg.github.com/OWNER
-@OWNER:registry=https://npm.pkg.github.com
-@OWNER:registry=https://npm.pkg.github.com
+$ npm login --scope=@OWNER --registry=https://HOSTNAME/_registry/npm/
+> Username: USERNAME
+> Password: TOKEN
+> Email: PUBLIC-EMAIL-ADDRESS
```
{% endif %}
diff --git a/translations/de-DE/data/reusables/enterprise-accounts/enterprise-accounts-billing.md b/translations/de-DE/data/reusables/enterprise-accounts/enterprise-accounts-billing.md
index ec69f9209f8b..0017ae20951a 100644
--- a/translations/de-DE/data/reusables/enterprise-accounts/enterprise-accounts-billing.md
+++ b/translations/de-DE/data/reusables/enterprise-accounts/enterprise-accounts-billing.md
@@ -1 +1 @@
-Enterprise-Konten sind momentan nicht verfügbar für Kunden von {% data variables.product.prodname_ghe_cloud %} und {% data variables.product.prodname_ghe_server %}, die mit Rechnung bezahlen. Die Abrechnung aller Organisations- und {% data variables.product.prodname_ghe_server %}-Instanzen, die mit Deinem Enterprise-Konto verbunden sind, wird in einer einzigen Rechnung zusammengefasst. Weitere Informationen zur Verwaltung Deines {% data variables.product.prodname_ghe_cloud %}-Abonnements findest Du auf „[Abonnement und Nutzung Deines Enterprise-Kontos anzeigen](/articles/viewing-the-subscription-and-usage-for-your-enterprise-account)." For more information about managing your {% data variables.product.prodname_ghe_server %} billing settings, see "[Managing billing for your enterprise](/admin/overview/managing-billing-for-your-enterprise)."
+Enterprise-Konten sind momentan nicht verfügbar für Kunden von {% data variables.product.prodname_ghe_cloud %} und {% data variables.product.prodname_ghe_server %}, die mit Rechnung bezahlen. Die Abrechnung aller Organisations- und {% data variables.product.prodname_ghe_server %}-Instanzen, die mit Deinem Enterprise-Konto verbunden sind, wird in einer einzigen Rechnung zusammengefasst.
diff --git a/translations/de-DE/data/reusables/enterprise_installation/hardware-considerations-all-platforms.md b/translations/de-DE/data/reusables/enterprise_installation/hardware-considerations-all-platforms.md
index 409adc8162a0..e1c8e17ba40c 100644
--- a/translations/de-DE/data/reusables/enterprise_installation/hardware-considerations-all-platforms.md
+++ b/translations/de-DE/data/reusables/enterprise_installation/hardware-considerations-all-platforms.md
@@ -1,4 +1,5 @@
- [Minimum requirements](#minimum-requirements)
+- [Beta features in {% data variables.product.prodname_ghe_server %} 2.22](#beta-features-in-github-enterprise-server-222)
- [Speicher](#storage)
- [CPU and memory](#cpu-and-memory)
@@ -6,23 +7,18 @@
We recommend different hardware configurations depending on the number of user licenses for {% data variables.product.product_location %}. If you provision more resources than the minimum requirements, your instance will perform and scale better.
-{% data reusables.enterprise_installation.hardware-rec-table %} For more information about adjusting resources for an existing instance, see "[Increasing storage capacity](/enterprise/admin/installation/increasing-storage-capacity)" and "[Increasing CPU or memory resources](/enterprise/admin/installation/increasing-cpu-or-memory-resources)."
-
-{% if currentVersion == "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
-
-If you enable the beta for {% data variables.product.prodname_actions %} on your instance, we recommend planning for additional capacity.
+{% data reusables.enterprise_installation.hardware-rec-table %}{% if currentVersion == "enterprise-server@2.22" or currentVersion == "github-ae@latest" %} If you enable the beta for {% data variables.product.prodname_actions %}, review the following requirements and recommendations.
- You must configure at least one runner for {% data variables.product.prodname_actions %} workflows. Weitere Informationen findest Du unter „[Informationen zu selbst-gehosteten Runnern](/actions/hosting-your-own-runners/about-self-hosted-runners)“.
- You must configure external blob storage. For more information, see "[Enabling {% data variables.product.prodname_actions %} and configuring storage](/enterprise/admin/github-actions/enabling-github-actions-and-configuring-storage)."
-
-The additional CPU and memory resources you need to provision for your instance depend on the number of workflows your users run concurrently, and the overall levels of activity for users, automations, and integrations.
-
-| Maximum jobs per minute | vCPUs | Arbeitsspeicher |
-|:----------------------- | -----:| ---------------:|
-| Light testing | 4 | 30.5 GB |
-| 25 | 8 | 61 GB |
-| 35 | 16 | 122 GB |
-| 100 | 32 | 244 GB |
+- You may need to configure additional CPU and memory resources. The additional resources you need to provision for {% data variables.product.prodname_actions %} depend on the number of workflows your users run concurrently, and the overall levels of activity for users, automations, and integrations.
+
+ | Maximum jobs per minute | Additional vCPUs | Additional memory |
+ |:----------------------- | ----------------:| -----------------:|
+ | Light testing | 4 | 30.5 GB |
+ | 25 | 8 | 61 GB |
+ | 35 | 16 | 122 GB |
+ | 100 | 32 | 244 GB |
{% endif %}
diff --git a/translations/de-DE/data/reusables/enterprise_installation/hardware-rec-table.md b/translations/de-DE/data/reusables/enterprise_installation/hardware-rec-table.md
index 82cc36e9c90c..776fb6a27809 100644
--- a/translations/de-DE/data/reusables/enterprise_installation/hardware-rec-table.md
+++ b/translations/de-DE/data/reusables/enterprise_installation/hardware-rec-table.md
@@ -1,6 +1,12 @@
{% if currentVersion == "enterprise-server@2.22" %}
-Minimum requirements for an instance with beta features enabled are **bold** in the following table. For more information, see "[Beta features in {% data variables.product.prodname_ghe_server %} 2.22](#beta-features-in-github-enterprise-server-222)." |{% endif %}
+{% note %}
+
+**Note**: If you enable beta features for {% data variables.product.prodname_ghe_server %} 2.22, your instance requires additional hardware resources. Minimum requirements for an instance with beta features enabled are **bold** in the following table. For more information about the features in beta, see "[Beta features in {% data variables.product.prodname_ghe_server %} 2.22](#beta-features-in-github-enterprise-server-222)."
+
+{% endnote %}
+|
+{% endif %}
| Benutzerlizenzen | vCPUs | Arbeitsspeicher | Attached-Storage | Root-Storage |
|:---------------------------------------------------------- | --------------------------------------------------------------------------------------------------------------------------------------:| ----------------------------------------------------------------------------------------------------------------------------------------------:| ----------------------------------------------------------------------------------------------------------------------------------------------:| ------------:|
| Test, Demo oder 10 Benutzer mit eingeschränkten Funktionen | 2{% if currentVersion == "enterprise-server@2.22" %}
or [**4**](#beta-features-in-github-enterprise-server-222){% endif %} | 16 GB{% if currentVersion == "enterprise-server@2.22" %}
or [**32 GB**](#beta-features-in-github-enterprise-server-222){% endif %} | 100 GB{% if currentVersion == "enterprise-server@2.22" %}
or [**150 GB**](#beta-features-in-github-enterprise-server-222){% endif %} | 200 GB |
@@ -9,8 +15,14 @@ Minimum requirements for an instance with beta features enabled are **bold** in
| 5000–8000 | 12{% if currentVersion == "enterprise-server@2.22" %}
or [**16**](#beta-features-in-github-enterprise-server-222){% endif %} | 96 GB | 750 GB | 200 GB |
| 8000–10000+ | 16{% if currentVersion == "enterprise-server@2.22" %}
or [**20**](#beta-features-in-github-enterprise-server-222){% endif %} | 128 GB{% if currentVersion == "enterprise-server@2.22" %}
or [**160 GB**](#beta-features-in-github-enterprise-server-222){% endif %} | 1000 GB | 200 GB |
+For more information about adjusting resources for an existing instance, see "[Increasing storage capacity](/enterprise/admin/installation/increasing-storage-capacity)" and "[Increasing CPU or memory resources](/enterprise/admin/installation/increasing-cpu-or-memory-resources)."
+
{% if currentVersion == "enterprise-server@2.22" %}
#### Beta features in {% data variables.product.prodname_ghe_server %} 2.22
-If you enable beta features in {% data variables.product.prodname_ghe_server %} 2.22, your instance requires additional hardware resources. For more information about the beta features, see the [release notes for the 2.22 series](https://enterprise.github.com/releases/series/2.22) on the {% data variables.product.prodname_enterprise %} website.{% endif %}
+You can sign up for beta features available in {% data variables.product.prodname_ghe_server %} 2.22 such as {% data variables.product.prodname_actions %}, {% data variables.product.prodname_registry %}, and {% data variables.product.prodname_code_scanning %}. For more information, see the [release notes for the 2.22 series](https://enterprise.github.com/releases/series/2.22#release-2.22.0) on the {% data variables.product.prodname_enterprise %} website.
+
+If you enable beta features for {% data variables.product.prodname_ghe_server %} 2.22, your instance requires additional hardware resources. For more information, see "[Minimum requirements](#minimum-requirements)".
+
+{% endif %}
diff --git a/translations/de-DE/data/reusables/enterprise_installation/image-urls-viewable-warning.md b/translations/de-DE/data/reusables/enterprise_installation/image-urls-viewable-warning.md
index 68c25df5ed76..2a26ad6d64f7 100644
--- a/translations/de-DE/data/reusables/enterprise_installation/image-urls-viewable-warning.md
+++ b/translations/de-DE/data/reusables/enterprise_installation/image-urls-viewable-warning.md
@@ -1,5 +1,5 @@
{% warning %}
-**Warnung:** Wenn Du einen Bildanhang zu einem Pull-Request- oder Issue-Kommentar hinzufügst, kann jeder die anonymisierte Bild-URL ohne Authentifizierung anzeigen, selbst wenn sich der Pull Request in einem privaten Repository befindet oder der private Modus aktiviert ist. Um vertraulicher Bilder zu schützen, stelle sie über ein privates Netzwerk oder einen Server bereit, der eine Authentifizierung vorschreibt.
+**Warning:** If you add an image attachment to a pull request or issue comment, anyone can view the anonymized image URL without authentication{% if enterpriseServerVersions contains currentVersion %}, even if the pull request is in a private repository, or if private mode is enabled. To prevent unauthorized access to the images, ensure that you restrict network access to the systems that serve the images, including {% data variables.product.product_location %}{% endif %}.{% if currentVersion == "github-ae@latest" %} To prevent unauthorized access to image URLs on {% data variables.product.product_name %}, consider restricting network traffic to your enterprise. For more information, see "[Restricting network traffic to your enterprise](/admin/configuration/restricting-network-traffic-to-your-enterprise)."{% endif %}
{% endwarning %}
diff --git a/translations/de-DE/data/reusables/gated-features/pages.md b/translations/de-DE/data/reusables/gated-features/pages.md
index e24925cc9877..31caf392d2d3 100644
--- a/translations/de-DE/data/reusables/gated-features/pages.md
+++ b/translations/de-DE/data/reusables/gated-features/pages.md
@@ -1 +1 @@
-{% data variables.product.prodname_pages %} is available in public repositories with {% data variables.product.prodname_free_user %} and {% data variables.product.prodname_free_team %} for organizations, and in public and private repositories with {% data variables.product.prodname_pro %}, {% data variables.product.prodname_team %}, {% data variables.product.prodname_ghe_cloud %},{% if currentVersion == "github-ae@latest" %} {% data variables.product.prodname_ghe_managed %},{% endif %} and {% data variables.product.prodname_ghe_server %}. {% if currentVersion == "free-pro-team@latest" %}{% data reusables.gated-features.more-info %}{% endif %}
+{% if currentVersion == "github-ae@latest" %}{% data variables.product.prodname_pages %} is available in internal and private repositories with {% data variables.product.prodname_ghe_managed %}. {% endif %}{% data variables.product.prodname_pages %} is available in public repositories with {% data variables.product.prodname_free_user %} and {% data variables.product.prodname_free_team %} for organizations, and in public and private repositories with {% data variables.product.prodname_pro %}, {% data variables.product.prodname_team %}, {% data variables.product.prodname_ghe_cloud %}, and {% data variables.product.prodname_ghe_server %}. {% if currentVersion == "free-pro-team@latest" %}{% data reusables.gated-features.more-info %}{% endif %}
diff --git a/translations/de-DE/data/reusables/gated-features/wikis.md b/translations/de-DE/data/reusables/gated-features/wikis.md
index 61f560896e02..d7611dc3638c 100644
--- a/translations/de-DE/data/reusables/gated-features/wikis.md
+++ b/translations/de-DE/data/reusables/gated-features/wikis.md
@@ -1 +1 @@
-Wikis are available in public repositories with {% data variables.product.prodname_free_user %} and {% data variables.product.prodname_free_team %} for organizations, and in public and private repositories with {% data variables.product.prodname_pro %}, {% data variables.product.prodname_team %}, {% data variables.product.prodname_ghe_cloud %},{% if currentVersion == "github-ae@latest" %} {% data variables.product.prodname_ghe_managed %},{% endif %} and {% data variables.product.prodname_ghe_server %}. {% if currentVersion == "free-pro-team@latest" %}{% data reusables.gated-features.more-info %}{% endif %}
+{% if currentVersion == "github-ae@latest" %}Wikis are available in internal and private repositories with {% data variables.product.prodname_ghe_managed %}.{% endif %} Wikis are available in public repositories with {% data variables.product.prodname_free_user %} and {% data variables.product.prodname_free_team %} for organizations, and in public and private repositories with {% data variables.product.prodname_pro %}, {% data variables.product.prodname_team %}, {% data variables.product.prodname_ghe_cloud %} and {% data variables.product.prodname_ghe_server %}. {% if currentVersion == "free-pro-team@latest" %}{% data reusables.gated-features.more-info %}{% endif %}
diff --git a/translations/de-DE/data/reusables/github-actions/self-hosted-runner-configure-runner-group-access.md b/translations/de-DE/data/reusables/github-actions/self-hosted-runner-configure-runner-group-access.md
index 97614dd5e5ae..9ac93498fa1e 100644
--- a/translations/de-DE/data/reusables/github-actions/self-hosted-runner-configure-runner-group-access.md
+++ b/translations/de-DE/data/reusables/github-actions/self-hosted-runner-configure-runner-group-access.md
@@ -1,2 +1,12 @@
-1. In the **Self-hosted runners** section of the settings page, click {% octicon "kebab-horizontal" aria-label="The horizontal kebab icon" %} next to the runner group you'd like to configure, then click **Edit name and [organization|repository] access**. 
-1. Select a new policy from the dropdown list, or modify the runner group name.
+1. In the **Self-hosted runners** section of the settings page, click {% octicon "kebab-horizontal" aria-label="The horizontal kebab icon" %} next to the runner group you'd like to configure, then click **Edit name and [organization|repository] access**.
+ 
+1. Modify your policy options, or change the runner group name.
+
+ {% warning %}
+
+ **Warning**
+ {% indented_data_reference site.data.reusables.github-actions.self-hosted-runner-security spaces=3 %}
+ For more information, see "[About self-hosted runners](/actions/hosting-your-own-runners/about-self-hosted-runners#self-hosted-runner-security-with-public-repositories)."
+
+ {% endwarning %}
+
diff --git a/translations/de-DE/data/reusables/notifications-v2/custom-notifications-beta.md b/translations/de-DE/data/reusables/notifications-v2/custom-notifications-beta.md
new file mode 100644
index 000000000000..dbcee80cfe1d
--- /dev/null
+++ b/translations/de-DE/data/reusables/notifications-v2/custom-notifications-beta.md
@@ -0,0 +1,9 @@
+{% if currentVersion == "free-pro-team@latest" %}
+
+{% note %}
+
+**Note:** Custom notifications are currently in beta and subject to change.
+
+{% endnote %}
+
+{% endif %}
diff --git a/translations/de-DE/data/reusables/notifications/outbound_email_tip.md b/translations/de-DE/data/reusables/notifications/outbound_email_tip.md
index 290cdf19f8a1..5a18d0418f4e 100644
--- a/translations/de-DE/data/reusables/notifications/outbound_email_tip.md
+++ b/translations/de-DE/data/reusables/notifications/outbound_email_tip.md
@@ -1,7 +1,9 @@
-{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
- {% tip %}
+{% if enterpriseServerVersions contains currentVersion %}
- Du wirst nur dann E-Mail-Benachrichtigungen erhalten, wenn der ausgehende E-Mail-Support auf {% data variables.product.product_location %} aktiviert ist. Für weitere Informationen kontaktiere Deinen Websiteadministrator.
+{% note %}
+
+**Note**: You'll only receive email notifications if outbound email support is enabled on {% data variables.product.product_location %}. Für weitere Informationen kontaktiere Deinen Websiteadministrator.
+
+{% endnote %}
- {% endtip %}
{% endif %}
diff --git a/translations/de-DE/data/reusables/notifications/shared_state.md b/translations/de-DE/data/reusables/notifications/shared_state.md
index 479d545eec64..8d5e1575850a 100644
--- a/translations/de-DE/data/reusables/notifications/shared_state.md
+++ b/translations/de-DE/data/reusables/notifications/shared_state.md
@@ -1,5 +1,5 @@
{% tip %}
-**Tipp:** Wenn Du sowohl Web- als auch E-Mail-Benachrichtigungen erhältst, kannst Du den Gelesen- oder Ungelesen-Status der Benachrichtigung automatisch synchronisieren, sodass Webbenachrichtigungen automatisch als gelesen markiert werden, sobald Du die entsprechende E-Mail-Benachrichtigung gelesen hast. To enable this sync, your email client must be able to view images from {% if currentVersion == "free-pro-team@latest" %}'`notifications@github.com`'{% else %}'the no-reply email address configured by your site administrator'{% endif %}.
+**Tipp:** Wenn Du sowohl Web- als auch E-Mail-Benachrichtigungen erhältst, kannst Du den Gelesen- oder Ungelesen-Status der Benachrichtigung automatisch synchronisieren, sodass Webbenachrichtigungen automatisch als gelesen markiert werden, sobald Du die entsprechende E-Mail-Benachrichtigung gelesen hast. To enable this sync, your email client must be able to view images from {% if currentVersion == "free-pro-team@latest" %}`notifications@github.com`{% else %}the `no-reply` email address {% if currentVersion == "github-ae@latest" %}for your {% data variables.product.product_name %} hostname{% elsif enterpriseServerVersions contains currentVersion %}for {% data variables.product.product_location %}, which your site administrator configures{% endif %}{% endif %}.
{% endtip %}
diff --git a/translations/de-DE/data/reusables/profile/profile-readme.md b/translations/de-DE/data/reusables/profile/profile-readme.md
index a19a3d4a30d3..7350ed57bd30 100644
--- a/translations/de-DE/data/reusables/profile/profile-readme.md
+++ b/translations/de-DE/data/reusables/profile/profile-readme.md
@@ -1 +1 @@
-If you add a README file to the root of a public repository with the same name as your username, that README will automatically appear on your profile page. You can edit your profile README with GitHub Flavored Markdown to create a personalized section on your profile.
+If you add a README file to the root of a public repository with the same name as your username, that README will automatically appear on your profile page. You can edit your profile README with {% data variables.product.company_short %} Flavored Markdown to create a personalized section on your profile. For more information, see "[Managing your profile README](/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme)."
diff --git a/translations/de-DE/data/reusables/repositories/administrators-can-disable-issues.md b/translations/de-DE/data/reusables/repositories/administrators-can-disable-issues.md
new file mode 100644
index 000000000000..1ab1ffc4b5d1
--- /dev/null
+++ b/translations/de-DE/data/reusables/repositories/administrators-can-disable-issues.md
@@ -0,0 +1 @@
+Repository administrators can disable issues for a repository. Weitere Informationen findest Du unter „[Issues deaktivieren](/github/managing-your-work-on-github/disabling-issues)."
diff --git a/translations/de-DE/data/reusables/repositories/desktop-fork.md b/translations/de-DE/data/reusables/repositories/desktop-fork.md
index dc7f4cb32848..07becae5625b 100644
--- a/translations/de-DE/data/reusables/repositories/desktop-fork.md
+++ b/translations/de-DE/data/reusables/repositories/desktop-fork.md
@@ -1 +1 @@
-Du kannst {% data variables.product.prodname_desktop %} verwenden, um ein Repository zu forken. Weitere Informationen findest Du unter „[Klonen und Forking von Repositorys von {% data variables.product.prodname_desktop %}](/desktop/contributing-to-projects/cloning-and-forking-repositories-from-github-desktop)."
+Du kannst {% data variables.product.prodname_desktop %} verwenden, um ein Repository zu forken. For more information, see "[Cloning and forking repositories from {% data variables.product.prodname_desktop %}](/desktop/contributing-to-projects/cloning-and-forking-repositories-from-github-desktop)."
diff --git a/translations/de-DE/data/reusables/repositories/you-can-fork.md b/translations/de-DE/data/reusables/repositories/you-can-fork.md
index c2de2edf8f60..06d179d94dce 100644
--- a/translations/de-DE/data/reusables/repositories/you-can-fork.md
+++ b/translations/de-DE/data/reusables/repositories/you-can-fork.md
@@ -1,3 +1,7 @@
-Du kannst jedes öffentliche Repository zu Deinem Benutzerkonto oder zu jeder Organisation forken, in der Du die Berechtigung zum Erstellen von Repositorys hast. Weitere Informationen finden Sie unter „[Berechtigungsebenen für eine Organisation](/articles/permission-levels-for-an-organization)".
+{% if currentVersion == "github-ae@latest" %}If the policies for your enterprise permit forking internal and private repositories, you{% else %}You{% endif %} can fork a repository to your user account or any organization where you have repository creation permissions. Weitere Informationen finden Sie unter „[Berechtigungsebenen für eine Organisation](/articles/permission-levels-for-an-organization)".
-Du kannst jedes private Repository, auf das Du Zugriff hast, zu Deinem Benutzerkonto oder zu jeder Organisation auf {% data variables.product.prodname_team %} oder {% data variables.product.prodname_enterprise %} forken, in denen Du die Berechtigung zum Erstellen von Repositorys hast. You cannot fork a private repository to an organization using {% data variables.product.prodname_free_team %}.{% if currentVersion == "free-pro-team@latest" %} For more information, see "[GitHub's products](/articles/githubs-products)."{% endif %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+If you have access to a private repository and the owner permits forking, you can fork the repository to your user account or any organization on {% if currentVersion == "free-pro-team@latest"%}{% data variables.product.prodname_team %}{% else %}{% data variables.product.product_location %}{% endif %} where you have repository creation permissions. {% if currentVersion == "free-pro-team@latest" %}You cannot fork a private repository to an organization using {% data variables.product.prodname_free_team %}. For more information, see "[GitHub's products](/articles/githubs-products)."{% endif %}
+
+{% endif %}
\ No newline at end of file
diff --git a/translations/de-DE/data/reusables/search/required_login.md b/translations/de-DE/data/reusables/search/required_login.md
index fc71fcd14b55..6d8598a94a38 100644
--- a/translations/de-DE/data/reusables/search/required_login.md
+++ b/translations/de-DE/data/reusables/search/required_login.md
@@ -1 +1 @@
-Du musst angemeldet sein, um über alle öffentlichen Repositorys hinweg nach Code suchen zu können.
+You must be signed into a user account on {% data variables.product.product_name %} to search for code across all public repositories.
diff --git a/translations/de-DE/data/reusables/search/syntax_tips.md b/translations/de-DE/data/reusables/search/syntax_tips.md
index 3236183c31ef..5f383b0bd0b3 100644
--- a/translations/de-DE/data/reusables/search/syntax_tips.md
+++ b/translations/de-DE/data/reusables/search/syntax_tips.md
@@ -1,7 +1,7 @@
{% tip %}
**Tips:**{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
- - Dieser Artikel enthält Beispielsuchen für die Website {% data variables.product.prodname_dotcom %}.com. Die gleichen Suchfilter kannst Du jedoch auch auf {% data variables.product.product_location %} verwenden.{% endif %}
+ - This article contains links to example searches on the {% data variables.product.prodname_dotcom_the_website %} website, but you can use the same search filters with {% data variables.product.product_name %}. In the linked example searches, replace `github.com` with the hostname for {% data variables.product.product_location %}.{% endif %}
- Eine Liste mit Suchsyntax, die Du jedem Qualifizierer hinzufügen kannst, um Deine Ergebnisse zu verbessern, findest Du unter „[Grundlagen der Suchsyntax](/articles/understanding-the-search-syntax)“.
- Schließe Suchbegriffe, die aus mehreren Wörtern bestehen, in Anführungszeichen ein. Möchtest Du beispielsweise nach Issues mit der Kennzeichnung „In progress“ suchen, gib `label:"in progress"` ein. Bei der Suche wird die Groß-/Kleinschreibung ignoriert.
diff --git a/translations/de-DE/data/reusables/search/you-can-search-globally.md b/translations/de-DE/data/reusables/search/you-can-search-globally.md
new file mode 100644
index 000000000000..7a15c0993a9b
--- /dev/null
+++ b/translations/de-DE/data/reusables/search/you-can-search-globally.md
@@ -0,0 +1 @@
+Du kannst global über {% data variables.product.product_name %} hinweg suchen oder Deine Suche auf ein bestimmtes Repositorys oder eine bestimmte Organisation beschränken.
diff --git a/translations/de-DE/data/reusables/ssh/add-ssh-key-to-ssh-agent-commandline.md b/translations/de-DE/data/reusables/ssh/add-ssh-key-to-ssh-agent-commandline.md
index af4760bd1725..415f86736de3 100644
--- a/translations/de-DE/data/reusables/ssh/add-ssh-key-to-ssh-agent-commandline.md
+++ b/translations/de-DE/data/reusables/ssh/add-ssh-key-to-ssh-agent-commandline.md
@@ -1,3 +1,3 @@
```shell
-$ ssh-add ~/.ssh/id_rsa
+$ ssh-add ~/.ssh/id_ed25519
```
diff --git a/translations/de-DE/data/reusables/ssh/add-ssh-key-to-ssh-agent.md b/translations/de-DE/data/reusables/ssh/add-ssh-key-to-ssh-agent.md
index 5320f1ead215..fb1ca5ec3428 100644
--- a/translations/de-DE/data/reusables/ssh/add-ssh-key-to-ssh-agent.md
+++ b/translations/de-DE/data/reusables/ssh/add-ssh-key-to-ssh-agent.md
@@ -1 +1 @@
-Wenn Du Deinen Schlüssel mit einem anderen Namen erstellt hast, oder wenn Du einen vorhandenen Schlüssel mit einem anderen Namen hinzufügst, ersetze *id_rsa* im Befehl durch den Namen Deiner Privatschlüssel-Datei.
+If you created your key with a different name, or if you are adding an existing key that has a different name, replace *id_ed25519* in the command with the name of your private key file.
diff --git a/translations/de-DE/data/variables/action_code_examples.yml b/translations/de-DE/data/variables/action_code_examples.yml
index e7c5decbd2f5..a5b26266eb0d 100644
--- a/translations/de-DE/data/variables/action_code_examples.yml
+++ b/translations/de-DE/data/variables/action_code_examples.yml
@@ -6,7 +6,7 @@
href: actions/starter-workflows
tags:
- official
- - workflows
+ - Workflow
-
title: Example services
description: Example workflows using service containers
@@ -64,7 +64,7 @@
- publishing
-
title: GitHub Project Automation+
- description: Automate GitHub Project cards with any webhook event.
+ description: Automate GitHub Project cards with any webhook event
languages: JavaScript
href: alex-page/github-project-automation-plus
tags:
@@ -131,8 +131,7 @@
- publishing
-
title: Label your Pull Requests auto-magically (using committed files)
- description: >-
- Github action to label your pull requests auto-magically (using committed files)
+ description: Github action to label your pull requests auto-magically (using committed files)
languages: 'TypeScript, Dockerfile, JavaScript'
href: Decathlon/pull-request-labeler-action
tags:
@@ -147,3 +146,191 @@
tags:
- Pull Request
- labels
+-
+ title: Get a list of file changes with a PR/Push
+ description: This action gives you will get outputs of the files that have changed in your repository
+ languages: 'TypeScript, Shell, JavaScript'
+ href: trilom/file-changes-action
+ tags:
+ - Workflow
+ - Repository
+-
+ title: Private actions in any workflow
+ description: Allows private GitHub Actions to be easily reused
+ languages: 'TypeScript, JavaScript, Shell'
+ href: InVisionApp/private-action-loader
+ tags:
+ - Workflow
+ - tools
+-
+ title: Label your issues using the issue's contents
+ description: A GitHub Action to automatically tag issues with labels and assignees
+ languages: 'JavaScript, TypeScript'
+ href: damccorm/tag-ur-it
+ tags:
+ - Workflow
+ - tools
+ - labels
+ - Issues (Lieferungen)
+-
+ title: Rollback a GitHub Release
+ description: A GitHub Action to rollback or delete a release
+ languages: 'JavaScript'
+ href: author/action-rollback
+ tags:
+ - Workflow
+ - veröffentlichungen
+-
+ title: Lock closed issues and Pull Requests
+ description: GitHub Action that locks closed issues and pull requests after a period of inactivity
+ languages: 'JavaScript'
+ href: dessant/lock-threads
+ tags:
+ - Issues (Lieferungen)
+ - pull requests
+ - Workflow
+-
+ title: Get Commit Difference Count Between Two Branches
+ description: This GitHub Action compares two branches and gives you the commit count between them
+ languages: 'JavaScript, Shell'
+ href: jessicalostinspace/commit-difference-action
+ tags:
+ - Commit
+ - Diff
+ - Workflow
+-
+ title: Generate Release Notes Based on Git References
+ description: GitHub Action to generate changelogs, and release notes
+ languages: 'JavaScript, Shell'
+ href: metcalfc/changelog-generator
+ tags:
+ - cicd
+ - release-notes
+ - Workflow
+ - changelog
+-
+ title: Enforce Policies on GitHub Repositories and Commits
+ description: Policy enforcement for your pipelines
+ languages: 'Go, Makefile, Dockerfile, Shell'
+ href: talos-systems/conform
+ tags:
+ - docker
+ - build-automation
+ - Workflow
+-
+ title: Auto Label Issue Based
+ description: Automatically label an issue based on the issue description
+ languages: 'TypeScript, JavaScript, Dockerfile'
+ href: Renato66/auto-label
+ tags:
+ - labels
+ - Workflow
+ - automation
+-
+ title: Update Configured GitHub Actions to the Latest Versions
+ description: CLI tool to check whehter all your actions are up-to-date or not
+ languages: 'C#, Inno Setup, PowerSHell, Shell'
+ href: fabasoad/ghacu
+ tags:
+ - versions
+ - cli
+ - Workflow
+-
+ title: Create Issue Branch
+ description: GitHub Action that automates the creation of issue branches
+ languages: 'JavaScript, Shell'
+ href: robvanderleek/create-issue-branch
+ tags:
+ - probot
+ - Issues (Lieferungen)
+ - labels
+-
+ title: Remove old artifacts
+ description: Customize artifact cleanup
+ languages: 'JavaScript, Shell'
+ href: c-hive/gha-remove-artifacts
+ tags:
+ - artifacts
+ - Workflow
+-
+ title: Sync Defined Files/Binaries to Wiki or External Repositories
+ description: GitHub Action to automatically sync changes to external repositories, like the wiki, for example
+ languages: 'Shell, Dockerfile'
+ href: kai-tub/external-repo-sync-action
+ tags:
+ - wiki
+ - sync
+ - Workflow
+-
+ title: Create/Update/Delete a GitHub Wiki page based on any file
+ description: Updates your GitHub wiki by using rsync, allowing for exclusion of files and directories and actual deletion of files
+ languages: 'Shell, Dockerfile'
+ href: Andrew-Chen-Wang/github-wiki-action
+ tags:
+ - wiki
+ - docker
+ - Workflow
+-
+ title: Prow GitHub Actions
+ description: Automation of policy enforcement, chat-ops, and automatic PR merging
+ languages: 'TypeScript, JavaScript'
+ href: jpmcb/prow-github-actions
+ tags:
+ - chat-ops
+ - prow
+ - Workflow
+-
+ title: Check GitHub Status in your Workflow
+ description: Check GitHub Status in your workflow
+ languages: 'TypeScript, JavaScript'
+ href: crazy-max/ghaction-github-status
+ tags:
+ - Status
+ - überwachung
+ - Workflow
+-
+ title: Manage labels on GitHub as code
+ description: GitHub Action to manage labels (create/rename/update/delete)
+ languages: 'TypeScript, JavaScript'
+ href: crazy-max/ghaction-github-labeler
+ tags:
+ - labels
+ - Workflow
+ - automation
+-
+ title: Distribute funding in free and open source projects
+ description: Continuous Distribution of funding to project contributors and dependencies
+ languages: 'Python, Docerfile, Shell, Ruby'
+ href: protontypes/libreselery
+ tags:
+ - sponsors
+ - funding
+ - Bezahlung
+-
+ title: Herald rules for GitHub
+ description: Add reviewers, subscribers, labels and assignees to your PR
+ languages: 'TypeScript, JavaScript'
+ href: gagoar/use-herald-action
+ tags:
+ - reviewers
+ - labels
+ - assignees
+ - Pull Request
+-
+ title: Codeowner validator
+ description: Ensures the correctness of your GitHub CODEOWNERS file, supports public and private GitHub repositories and also GitHub Enterprise installations
+ languages: 'Go, Shell, Makefile, Docerfile'
+ href: mszostok/codeowners-validator
+ tags:
+ - codeowners
+ - validate
+ - Workflow
+-
+ title: Copybara Action
+ description: Move and transform code between repositories (ideal to maintain several repos from one monorepo)
+ languages: 'TypeScript, JavaScript, Shell'
+ href: olivr/copybara-action
+ tags:
+ - monorepo
+ - copybara
+ - Workflow
diff --git a/translations/ja-JP/content/actions/hosting-your-own-runners/managing-access-to-self-hosted-runners-using-groups.md b/translations/ja-JP/content/actions/hosting-your-own-runners/managing-access-to-self-hosted-runners-using-groups.md
index 7c7798d47ae0..d1641858552a 100644
--- a/translations/ja-JP/content/actions/hosting-your-own-runners/managing-access-to-self-hosted-runners-using-groups.md
+++ b/translations/ja-JP/content/actions/hosting-your-own-runners/managing-access-to-self-hosted-runners-using-groups.md
@@ -1,6 +1,6 @@
---
-title: グループを使用してセルフホストランナーへのアクセスを管理する
-intro: ポリシーを使用して、Organization または Enterprise に追加されたセルフホストランナーへのアクセスを制限できます。
+title: Managing access to self-hosted runners using groups
+intro: You can use policies to limit access to self-hosted runners that have been added to an organization or enterprise.
redirect_from:
- /actions/hosting-your-own-runners/managing-access-to-self-hosted-runners
versions:
@@ -8,84 +8,105 @@ versions:
enterprise-server: '>=2.22'
---
-{% data variables.product.prodname_actions %} の支払いを管理する
-{% data variables.product.prodname_dotcom %}は、macOSランナーのホストに[MacStadium](https://www.macstadium.com/)を使用しています。
+{% data reusables.actions.enterprise-beta %}
+{% data reusables.actions.enterprise-github-hosted-runners %}
-### セルフホストランナーのグループについて
+### About self-hosted runner groups
{% if currentVersion == "free-pro-team@latest" %}
{% note %}
-**注釈:** すべての Organization には、単一のデフォルトのセルフホストランナーグループがあります。 追加のセルフホストランナーグループの作成と管理は、Enterprise アカウント、および Enterprise アカウントが所有する Organization でのみ使用できます。
+**Note:** All organizations have a single default self-hosted runner group. Creating and managing additional self-hosted runner groups is only available to enterprise accounts, and for organizations owned by an enterprise account.
{% endnote %}
{% endif %}
-セルフホストランナーグループは、Organization レベルおよび Enterprise レベルでセルフホストランナーへのアクセスを制御するために使用されます。 Enterprise の管理者は、Enterprise 内のどの Organization がランナーグループにアクセスできるかを制御するアクセスポリシーを設定できます。 Organization の管理者は、Organization 内のどのリポジトリがランナーグループにアクセスできるかを制御するアクセスポリシーを設定できます。
+Self-hosted runner groups are used to control access to self-hosted runners at the organization and enterprise level. Enterprise admins can configure access policies that control which organizations in an enterprise have access to the runner group. Organization admins can configure access policies that control which repositories in an organization have access to the runner group.
-Enterprise の管理者が Organization にランナーグループへのアクセスを許可すると、Organization の管理者は、Organization のセルフホストランナー設定にリストされたランナーグループを表示できます。 Organization の管理者は、追加の詳細なリポジトリアクセスポリシーを Enterprise ランナーグループに割り当てることができます。
+When an enterprise admin grants an organization access to a runner group, organization admins can see the runner group listed in the organization's self-hosted runner settings. The organizations admins can then assign additional granular repository access policies to the enterprise runner group.
-新しいランナーが作成されると、それらは自動的にデフォルトグループに割り当てられます。 ランナーは一度に1つのグループにのみ参加できます。 ランナーはデフォルトグループから別のグループに移動できます。 詳しい情報については、「[セルフホストランナーをグループに移動する](#moving-a-self-hosted-runner-to-a-group)」を参照してください。
+When new runners are created, they are automatically assigned to the default group. Runners can only be in one group at a time. You can move runners from the default group to another group. For more information, see "[Moving a self-hosted runner to a group](#moving-a-self-hosted-runner-to-a-group)."
-### Organization のセルフホストランナーグループを作成する
+### Creating a self-hosted runner group for an organization
-すべての Organization には、単一のデフォルトのセルフホストランナーグループがあります。 Enterprise アカウント内の Organization は、追加のセルフホストグループを作成できます。 Organization の管理者は、個々のリポジトリにランナーグループへのアクセスを許可できます。
+All organizations have a single default self-hosted runner group. Organizations within an enterprise account can create additional self-hosted groups. Organization admins can allow individual repositories access to a runner group.
-セルフホストランナーは、作成時にデフォルトグループに自動的に割り当てられ、一度に 1 つのグループのメンバーになることができます。 ランナーはデフォルトグループから作成した任意のグループに移動できます。
+Self-hosted runners are automatically assigned to the default group when created, and can only be members of one group at a time. You can move a runner from the default group to any group you create.
-グループを作成する場合、ランナーグループにアクセスできるリポジトリを定義するポリシーを選択する必要があります。 ランナーグループを設定して、リポジトリの特定のリスト、すべてのプライベートリポジトリ、または Organization 内のすべてのリポジトリにアクセスできます。
+When creating a group, you must choose a policy that defines which repositories have access to the runner group.
{% data reusables.organizations.navigate-to-org %}
{% data reusables.organizations.org_settings %}
{% data reusables.organizations.settings-sidebar-actions %}
-1. [**Self-hosted runners**] セクションで、[**Add new**] をクリックし、次に [**New group**] をクリックします。
+1. In the **Self-hosted runners** section, click **Add new**, and then **New group**.
- 
-1. ランナーグループの名前を入力し、[**Repository access**] ドロップダウンリストからアクセスポリシーを選択します。
+ 
+1. Enter a name for your runner group, and assign a policy for repository access.
- 
-1. [**Save group**] をクリックしてグループを作成し、ポリシーを適用します。
+ {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %} You can configure a runner group to be accessible to a specific list of repositories, or to all repositories in the organization. By default, public repositories can't access runners in a runner group, but you can use the **Allow public repositories** option to override this.{% else if currentVersion == "enterprise-server@2.22"%}You can configure a runner group to be accessible to a specific list of repositories, all private repositories, or all repositories in the organization.{% endif %}
-### Enterprise のセルフホストランナーグループを作成する
+ {% warning %}
-Enterprise は、セルフホストランナーをグループに追加して、アクセス管理を行うことができます。 Enterprise は、Enterprise アカウント内の特定の Organization がアクセスできるセルフホストランナーのグループを作成できます。 Organization の管理者は、追加の詳細なリポジトリアクセスポリシーを Enterprise ランナーグループに割り当てることができます。
+ **Warnung**
+ {% indented_data_reference site.data.reusables.github-actions.self-hosted-runner-security spaces=3 %}
+ Weitere Informationen findest Du unter „[Informationen zu selbst-gehosteten Runnern](/actions/hosting-your-own-runners/about-self-hosted-runners#self-hosted-runner-security-with-public-repositories)“.
-セルフホストランナーは、作成時にデフォルトグループに自動的に割り当てられ、一度に 1 つのグループのメンバーになることができます。 登録処理中にランナーを特定のグループに割り当てることも、後でランナーをデフォルトグループからカスタムグループに移動することもできます。
+ {% endwarning %}
-グループを作成するときは、Enterprise 内のすべての Organization にアクセスを付与するポリシーを選択するか、特定の Organization を選択する必要があります。
+ 
+1. Click **Save group** to create the group and apply the policy.
+
+### Creating a self-hosted runner group for an enterprise
+
+Enterprises can add their self-hosted runners to groups for access management. Enterprises can create groups of self-hosted runners that are accessible to specific organizations in the enterprise account. Organization admins can then assign additional granular repository access policies to the enterprise runner groups.
+
+Self-hosted runners are automatically assigned to the default group when created, and can only be members of one group at a time. You can assign the runner to a specific group during the registration process, or you can later move the runner from the default group to a custom group.
+
+When creating a group, you must choose a policy that defines which organizations have access to the runner group.
{% data reusables.enterprise-accounts.access-enterprise %}
{% data reusables.enterprise-accounts.policies-tab %}
{% data reusables.enterprise-accounts.actions-tab %}
-1. [**Self-hosted runners**] タブをクリックします。
-1. [**Add new**] をクリックしてから、[**New group**] をクリックします。
+1. Click the **Self-hosted runners** tab.
+1. Click **Add new**, and then **New group**.
+
+ 
+1. Enter a name for your runner group, and assign a policy for organization access.
- 
-1. ランナーグループの名前を入力し、[**Organization access**] ドロップダウンリストからアクセスポリシーを選択します。
+ {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %} You can configure a runner group to be accessible to a specific list of organizations, or all organizations in the enterprise. By default, public repositories can't access runners in a runner group, but you can use the **Allow public repositories** option to override this.{% else if currentVersion == "enterprise-server@2.22"%}You can configure a runner group to be accessible to all organizations in the enterprise or choose specific organizations.{% endif %}
- 
-1. [**Save group**] をクリックしてグループを作成し、ポリシーを適用します。
+ {% warning %}
-### セルフホストランナーグループのアクセスポリシーを変更する
+ **Warnung**
+ {% indented_data_reference site.data.reusables.github-actions.self-hosted-runner-security spaces=3 %}
+ Weitere Informationen findest Du unter „[Informationen zu selbst-gehosteten Runnern](/actions/hosting-your-own-runners/about-self-hosted-runners#self-hosted-runner-security-with-public-repositories)“.
-ランナーグループのアクセスポリシーを更新したり、ランナーグループの名前を変更したりすることができます。
+ {% endwarning %}
+
+ 
+1. Click **Save group** to create the group and apply the policy.
+
+### Changing the access policy of a self-hosted runner group
+
+You can update the access policy of a runner group, or rename a runner group.
{% data reusables.github-actions.self-hosted-runner-configure-runner-group-access %}
-### セルフホストランナーをグループに移動する
+### Moving a self-hosted runner to a group
+
+New self-hosted runners are automatically assigned to the default group, and can then be moved to another group.
-新しいセルフホストランナーは自動的にデフォルトグループに割り当てられ、その後、別のグループに移動できます。
+1. In the **Self-hosted runners** section of the settings page, locate the current group of the runner you want to move group and expand the list of group members. 
+1. Select the checkbox next to the self-hosted runner, and then click **Move to group** to see the available destinations. 
+1. To move the runner, click on the destination group. 
-1. 設定ページの [**Self-hosted runners**] セクションで、グループを移動するランナーの現在のグループを見つけ、グループメンバーのリストを展開します。 
-1. セルフホストランナーの横にあるチェックボックスを選択し、[**Move to group**] をクリックして、利用可能な移動先を確認します。 
-1. 移動先のグループをクリックして、ランナーを移動します。 
+### Removing a self-hosted runner group
-### セルフホストランナーグループを削除する
+Self-hosted runners are automatically returned to the default group when their group is removed.
-セルフホストランナーは、グループが削除されると自動的にデフォルトグループに戻ります。
+1. In the **Self-hosted runners** section of the settings page, locate the group you want to delete, and click the {% octicon "kebab-horizontal" aria-label="The horizontal kebab icon" %} button. 
-1. 設定ページの [**Self-hosted runners**] セクションで、削除するグループを見つけて、{% octicon "kebab-horizontal" aria-label="The horizontal kebab icon" %} ボタンをクリックします。 
+1. To remove the group, click **Remove group**. 
-1. グループを削除するには、[**Remove group**] をクリックします。 
+1. Review the confirmation prompts, and click **Remove this runner group**.
-1. 確認プロンプトを確認し、[**Remove this runner group**] をクリックします。
diff --git a/translations/ja-JP/content/actions/reference/context-and-expression-syntax-for-github-actions.md b/translations/ja-JP/content/actions/reference/context-and-expression-syntax-for-github-actions.md
index 04696f10e6c3..a357a3a933f4 100644
--- a/translations/ja-JP/content/actions/reference/context-and-expression-syntax-for-github-actions.md
+++ b/translations/ja-JP/content/actions/reference/context-and-expression-syntax-for-github-actions.md
@@ -59,7 +59,7 @@ env:
| ---------- | -------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| `github` | `オブジェクト` | ワークフロー実行に関する情報。 詳しい情報については、「[`github` コンテキスト](#github-context)」を参照してください。 |
| `env` | `オブジェクト` | ワークフロー、ジョブ、ステップで設定された環境変数が含まれます。 詳しい情報については[`env`コンテキスト](#env-context)を参照してください。 |
-| `ジョブ` | `オブジェクト` | 現在実行中のジョブに関する情報。 詳しい情報については、「[`job` コンテキスト](#job-context)」を参照してください。 |
+| `job` | `オブジェクト` | 現在実行中のジョブに関する情報。 詳しい情報については、「[`job` コンテキスト](#job-context)」を参照してください。 |
| `steps` | `オブジェクト` | このジョブで実行されているステップに関する情報。 詳しい情報については、「[`steps` コンテキスト](#steps-context)」を参照してください。 |
| `runner` | `オブジェクト` | 現在のジョブを実行している runner に関する情報。 詳しい情報については[`runner`コンテキスト](#runner-context)を参照してください。 |
| `secrets` | `オブジェクト` | シークレットへのアクセスを有効にします。 シークレットに関する詳しい情報については、「[暗号化されたシークレットの作成と利用](/actions/automating-your-workflow-with-github-actions/creating-and-using-encrypted-secrets)」を参照してください。 |
diff --git a/translations/ja-JP/content/actions/reference/events-that-trigger-workflows.md b/translations/ja-JP/content/actions/reference/events-that-trigger-workflows.md
index 1250ae66e5e9..56f38cc17c08 100644
--- a/translations/ja-JP/content/actions/reference/events-that-trigger-workflows.md
+++ b/translations/ja-JP/content/actions/reference/events-that-trigger-workflows.md
@@ -705,6 +705,8 @@ on:
{% data reusables.developer-site.pull_request_forked_repos_link %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %}
+
#### `pull_request_target`
@@ -728,6 +730,8 @@ on: pull_request_target
```
+{% endif %}
+
#### `push`
@@ -858,6 +862,8 @@ on:
```
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %}
+
#### `workflow_run`
@@ -886,6 +892,8 @@ on:
```
+{% endif %}
+
### 個人アクセストークンを使った新しいワークフローのトリガー
diff --git a/translations/ja-JP/content/actions/reference/workflow-syntax-for-github-actions.md b/translations/ja-JP/content/actions/reference/workflow-syntax-for-github-actions.md
index b7d6cd8a76fb..5c1a003c3fa1 100644
--- a/translations/ja-JP/content/actions/reference/workflow-syntax-for-github-actions.md
+++ b/translations/ja-JP/content/actions/reference/workflow-syntax-for-github-actions.md
@@ -876,6 +876,37 @@ strategy:
{% endnote %}
+##### Using environment variables in a matrix
+
+You can add custom environment variables for each test combination by using `include` with `env`. You can then refer to the custom environment variables in a later step.
+
+In this example, the matrix entries for `node-version` are each configured to use different values for the `site` and `datacenter` environment variables. The `Echo site details` step then uses {% raw %}`env: ${{ matrix.env }}`{% endraw %} to refer to the custom variables:
+
+{% raw %}
+```yaml
+name: Node.js CI
+on: [push]
+jobs:
+ build:
+ runs-on: ubuntu-latest
+ strategy:
+ matrix:
+ include:
+ - node-version: 10.x
+ site: "prod"
+ datacenter: "site-a"
+ - node-version: 12.x
+ site: "dev"
+ datacenter: "site-b"
+ steps:
+ - name: Echo site details
+ env:
+ SITE: ${{ matrix.site }}
+ DATACENTER: ${{ matrix.datacenter }}
+ run: echo $SITE $DATACENTER
+```
+{% endraw %}
+
### **`jobs..strategy.fail-fast`**
`true`に設定すると、いずれかの`matrix`ジョブが失敗した場合に{% data variables.product.prodname_dotcom %}は進行中のジョブをすべてキャンセルします。 デフォルト: `true`
diff --git a/translations/ja-JP/content/admin/configuration/command-line-utilities.md b/translations/ja-JP/content/admin/configuration/command-line-utilities.md
index 185cf4279b67..12267d4b63c2 100644
--- a/translations/ja-JP/content/admin/configuration/command-line-utilities.md
+++ b/translations/ja-JP/content/admin/configuration/command-line-utilities.md
@@ -84,7 +84,7 @@ $ ghe-config -l
API レート制限からユーザのリストを除外できます。 詳しい情報については、「[REST API のリソース](/rest/overview/resources-in-the-rest-api#rate-limiting)」を参照してください。
``` shell
-$ ghe-config app.github.rate_limiting_exempt_users "hubot github-actions"
+$ ghe-config app.github.rate-limiting-exempt-users "hubot github-actions"
# ユーザーの hubot と github-actions をレート制限から除外する
```
{% endif %}
diff --git a/translations/ja-JP/content/admin/enterprise-management/configuring-high-availability-replication-for-a-cluster.md b/translations/ja-JP/content/admin/enterprise-management/configuring-high-availability-replication-for-a-cluster.md
index 9ba73f331eb7..9526a3e73fa0 100644
--- a/translations/ja-JP/content/admin/enterprise-management/configuring-high-availability-replication-for-a-cluster.md
+++ b/translations/ja-JP/content/admin/enterprise-management/configuring-high-availability-replication-for-a-cluster.md
@@ -57,32 +57,36 @@ Before you define a secondary datacenter for your passive nodes, ensure that you
mysql-master = HOSTNAME
redis-master = HOSTNAME
primary-datacenter = default
- ```
+ ```
- Optionally, change the name of the primary datacenter to something more descriptive or accurate by editing the value of `primary-datacenter`.
4. {% data reusables.enterprise_clustering.configuration-file-heading %} 各ノードの見出しの下に、新しいキー/値ペアのペアを追加して、ノードをデータセンターに割り当てます。 Use the same value as `primary-datacenter` from step 3 above. For example, if you want to use the default name (`default`), add the following key-value pair to the section for each node.
- datacenter = default
+ ```
+ datacenter = default
+ ```
When you're done, the section for each node in the cluster configuration file should look like the following example. {% data reusables.enterprise_clustering.key-value-pair-order-irrelevant %}
- ```shell
- [cluster "HOSTNAME"]
- datacenter = default
- hostname = HOSTNAME
- ipv4 = IP ADDRESS
+ ```shell
+ [cluster "HOSTNAME"]
+ datacenter = default
+ hostname = HOSTNAME
+ ipv4 = IP ADDRESS
+ ...
...
- ...
- ```
+ ```
- {% note %}
+ {% note %}
- **Note**: If you changed the name of the primary datacenter in step 3, find the `consul-datacenter` key-value pair in the section for each node and change the value to the renamed primary datacenter. For example, if you named the primary datacenter `primary`, use the following key-value pair for each node.
+ **Note**: If you changed the name of the primary datacenter in step 3, find the `consul-datacenter` key-value pair in the section for each node and change the value to the renamed primary datacenter. For example, if you named the primary datacenter `primary`, use the following key-value pair for each node.
- consul-datacenter = primary
+ ```
+ consul-datacenter = primary
+ ```
- {% endnote %}
+ {% endnote %}
{% data reusables.enterprise_clustering.apply-configuration %}
@@ -103,31 +107,37 @@ For an example configuration, see "[Example configuration](#example-configuratio
1. クラスタ内のノードごとに、同じバージョンの {% data variables.product.prodname_ghe_server %} を実行して、同じ仕様で一致する仮想マシンをプロビジョニングします。 Note the IPv4 address and hostname for each new cluster node. For more information, see "[Prerequisites](#prerequisites)."
- {% note %}
+ {% note %}
- **Note**: If you're reconfiguring high availability after a failover, you can use the old nodes from the primary datacenter instead.
+ **Note**: If you're reconfiguring high availability after a failover, you can use the old nodes from the primary datacenter instead.
- {% endnote %}
+ {% endnote %}
{% data reusables.enterprise_clustering.ssh-to-a-node %}
3. Back up your existing cluster configuration.
-
- cp /data/user/common/cluster.conf ~/$(date +%Y-%m-%d)-cluster.conf.backup
+
+ ```
+ cp /data/user/common/cluster.conf ~/$(date +%Y-%m-%d)-cluster.conf.backup
+ ```
4. Create a copy of your existing cluster configuration file in a temporary location, like _/home/admin/cluster-passive.conf_. Delete unique key-value pairs for IP addresses (`ipv*`), UUIDs (`uuid`), and public keys for WireGuard (`wireguard-pubkey`).
-
- grep -Ev "(?:|ipv|uuid|vpn|wireguard\-pubkey)" /data/user/common/cluster.conf > ~/cluster-passive.conf
+
+ ```
+ grep -Ev "(?:|ipv|uuid|vpn|wireguard\-pubkey)" /data/user/common/cluster.conf > ~/cluster-passive.conf
+ ```
5. Remove the `[cluster]` section from the temporary cluster configuration file that you copied in the previous step.
-
- git config -f ~/cluster-passive.conf --remove-section cluster
+
+ ```
+ git config -f ~/cluster-passive.conf --remove-section cluster
+ ```
6. Decide on a name for the secondary datacenter where you provisioned your passive nodes, then update the temporary cluster configuration file with the new datacenter name. Replace `SECONDARY` with the name you choose.
```shell
- sed -i 's/datacenter = default/datacenter = SECONDARY/g' ~/cluster-passive.conf
- ```
+ sed -i 's/datacenter = default/datacenter = SECONDARY/g' ~/cluster-passive.conf
+ ```
7. Decide on a pattern for the passive nodes' hostnames.
@@ -140,7 +150,7 @@ For an example configuration, see "[Example configuration](#example-configuratio
8. Open the temporary cluster configuration file from step 3 in a text editor. For example, you can use Vim.
```shell
- sudo vim ~/cluster-passive.conf
+ sudo vim ~/cluster-passive.conf
```
9. In each section within the temporary cluster configuration file, update the node's configuration. {% data reusables.enterprise_clustering.configuration-file-heading %}
@@ -150,37 +160,37 @@ For an example configuration, see "[Example configuration](#example-configuratio
- Add a new key-value pair, `replica = enabled`.
```shell
- [cluster "NEW PASSIVE NODE HOSTNAME"]
+ [cluster "NEW PASSIVE NODE HOSTNAME"]
+ ...
+ hostname = NEW PASSIVE NODE HOSTNAME
+ ipv4 = NEW PASSIVE NODE IPV4 ADDRESS
+ replica = enabled
+ ...
...
- hostname = NEW PASSIVE NODE HOSTNAME
- ipv4 = NEW PASSIVE NODE IPV4 ADDRESS
- replica = enabled
- ...
- ...
```
10. Append the contents of the temporary cluster configuration file that you created in step 4 to the active configuration file.
```shell
- cat ~/cluster-passive.conf >> /data/user/common/cluster.conf
- ```
+ cat ~/cluster-passive.conf >> /data/user/common/cluster.conf
+ ```
11. Designate the primary MySQL and Redis nodes in the secondary datacenter. Replace `REPLICA MYSQL PRIMARY HOSTNAME` and `REPLICA REDIS PRIMARY HOSTNAME` with the hostnames of the passives node that you provisioned to match your existing MySQL and Redis primaries.
```shell
- git config -f /data/user/common/cluster.conf cluster.mysql-master-replica REPLICA MYSQL PRIMARY HOSTNAME
- git config -f /data/user/common/cluster.conf cluster.redis-master-replica REPLICA REDIS PRIMARY HOSTNAME
- ```
+ git config -f /data/user/common/cluster.conf cluster.mysql-master-replica REPLICA MYSQL PRIMARY HOSTNAME
+ git config -f /data/user/common/cluster.conf cluster.redis-master-replica REPLICA REDIS PRIMARY HOSTNAME
+ ```
12. Enable MySQL to fail over automatically when you fail over to the passive replica nodes.
```shell
- git config -f /data/user/common/cluster.conf cluster.mysql-auto-failover true
+ git config -f /data/user/common/cluster.conf cluster.mysql-auto-failover true
```
- {% warning %}
+ {% warning %}
- **Warning**: Review your cluster configuration file before proceeding.
+ **Warning**: Review your cluster configuration file before proceeding.
- In the top-level `[cluster]` section, ensure that the values for `mysql-master-replica` and `redis-master-replica` are the correct hostnames for the passive nodes in the secondary datacenter that will serve as the MySQL and Redis primaries after a failover.
- In each section for an active node named `[cluster "ACTIVE NODE HOSTNAME"]`, double-check the following key-value pairs.
@@ -194,9 +204,9 @@ For an example configuration, see "[Example configuration](#example-configuratio
- `replica` should be configured as `enabled`.
- Take the opportunity to remove sections for offline nodes that are no longer in use.
- To review an example configuration, see "[Example configuration](#example-configuration)."
+ To review an example configuration, see "[Example configuration](#example-configuration)."
- {% endwarning %}
+ {% endwarning %}
13. Initialize the new cluster configuration. {% data reusables.enterprise.use-a-multiplexer %}
@@ -207,7 +217,7 @@ For an example configuration, see "[Example configuration](#example-configuratio
14. 初期化が完了すると、{% data variables.product.prodname_ghe_server %} は次のメッセージを表示します。
```shell
- Finished cluster initialization
+ Finished cluster initialization
```
{% data reusables.enterprise_clustering.apply-configuration %}
@@ -293,20 +303,28 @@ Initial replication between the active and passive nodes in your cluster takes t
{% data variables.product.prodname_ghe_server %} 管理シェルから利用できるコマンドラインツールを使用して、クラスタ内の任意のノードの進行状況を監視できます。 For more information about the administrative shell, see "[Accessing the administrative shell (SSH)](/enterprise/admin/configuration/accessing-the-administrative-shell-ssh)."
- Monitor replication of databases:
-
- /usr/local/share/enterprise/ghe-cluster-status-mysql
+
+ ```
+ /usr/local/share/enterprise/ghe-cluster-status-mysql
+ ```
- Monitor replication of repository and Gist data:
-
- ghe-spokes status
+
+ ```
+ ghe-spokes status
+ ```
- Monitor replication of attachment and LFS data:
-
- ghe-storage replication-status
+
+ ```
+ ghe-storage replication-status
+ ```
- Monitor replication of Pages data:
-
- ghe-dpages replication-status
+
+ ```
+ ghe-dpages replication-status
+ ```
You can use `ghe-cluster-status` to review the overall health of your cluster. For more information, see "[Command-line utilities](/enterprise/admin/configuration/command-line-utilities#ghe-cluster-status)."
diff --git a/translations/ja-JP/content/admin/enterprise-management/increasing-storage-capacity.md b/translations/ja-JP/content/admin/enterprise-management/increasing-storage-capacity.md
index a65808fa90a2..c0e3e9e12a0b 100644
--- a/translations/ja-JP/content/admin/enterprise-management/increasing-storage-capacity.md
+++ b/translations/ja-JP/content/admin/enterprise-management/increasing-storage-capacity.md
@@ -20,6 +20,8 @@ versions:
{% endnote %}
+#### Minimum requirements
+
{% data reusables.enterprise_installation.hardware-rec-table %}
### データパーティションサイズの増加
diff --git a/translations/ja-JP/content/admin/installation/installing-github-enterprise-server-on-google-cloud-platform.md b/translations/ja-JP/content/admin/installation/installing-github-enterprise-server-on-google-cloud-platform.md
index 091e0dce1292..952600434eed 100644
--- a/translations/ja-JP/content/admin/installation/installing-github-enterprise-server-on-google-cloud-platform.md
+++ b/translations/ja-JP/content/admin/installation/installing-github-enterprise-server-on-google-cloud-platform.md
@@ -26,14 +26,14 @@ Google Cloud Platformde{% data variables.product.product_location %}を起動す
{% data variables.product.prodname_ghe_server %} は、次の Google Compute Engine (GCE) マシンタイプでサポートされています。 詳しい情報については[Google Cloud Platformのマシンタイプの記事](https://cloud.google.com/compute/docs/machine-types)を参照してください。
-| | ハイメモリ |
-| | ------------- |
-| | n1-highmem-4 |
-| | n1-highmem-8 |
-| | n1-highmem-16 |
-| | n1-highmem-32 |
-| | n1-highmem-64 |
-| | n1-highmem-96 |
+| ハイメモリ |
+| ------------- |
+| n1-highmem-4 |
+| n1-highmem-8 |
+| n1-highmem-16 |
+| n1-highmem-32 |
+| n1-highmem-64 |
+| n1-highmem-96 |
#### 推奨マシンタイプ
@@ -54,7 +54,7 @@ Google Cloud Platformde{% data variables.product.product_location %}を起動す
1. [gcloud compute](https://cloud.google.com/compute/docs/gcloud-compute/)コマンドラインツールを使用して、パブリックな {% data variables.product.prodname_ghe_server %} イメージを一覧表示します。
```shell
$ gcloud compute images list --project github-enterprise-public --no-standard-images
- ```
+ ```
2. {% data variables.product.prodname_ghe_server %} の最新の GCE イメージのイメージ名をメモしておきます。
@@ -63,18 +63,18 @@ Google Cloud Platformde{% data variables.product.product_location %}を起動す
GCE 仮想マシンは、ファイアウォールが存在するネットワークのメンバーとして作成されます。 {% data variables.product.prodname_ghe_server %} VMに関連付けられているネットワークの場合、下記の表に一覧表示されている必要なポートを許可するようにファイアウォールを設定する必要があります。 Google Cloud Platform でのファイアウォールルールに関する詳しい情報については、Google ガイドの「[ファイアウォールルールの概要](https://cloud.google.com/vpc/docs/firewalls)」を参照してください。
1. gcloud compute コマンドラインツールを使用して、ネットワークを作成します。 詳しい情報については、Google ドキュメンテーションの「[gcloud compute networks create](https://cloud.google.com/sdk/gcloud/reference/compute/networks/create)」を参照してください。
- ```shell
- $ gcloud compute networks create NETWORK-NAME --subnet-mode auto
- ```
+ ```shell
+ $ gcloud compute networks create NETWORK-NAME --subnet-mode auto
+ ```
2. 下記の表にある各ポートに関するファイアウォールルールを作成します。 詳しい情報については、Googleドキュメンテーションの「[gcloud compute firewall-rules](https://cloud.google.com/sdk/gcloud/reference/compute/firewall-rules/)」を参照してください。
- ```shell
- $ gcloud compute firewall-rules create RULE-NAME \
- --network NETWORK-NAME \
- --allow tcp:22,tcp:25,tcp:80,tcp:122,udp:161,tcp:443,udp:1194,tcp:8080,tcp:8443,tcp:9418,icmp
- ```
- 次の表に、必要なポートと各ポートの使用目的を示します。
+ ```shell
+ $ gcloud compute firewall-rules create RULE-NAME \
+ --network NETWORK-NAME \
+ --allow tcp:22,tcp:25,tcp:80,tcp:122,udp:161,tcp:443,udp:1194,tcp:8080,tcp:8443,tcp:9418,icmp
+ ```
+ 次の表に、必要なポートと各ポートの使用目的を示します。
- {% data reusables.enterprise_installation.necessary_ports %}
+ {% data reusables.enterprise_installation.necessary_ports %}
### スタティックIPの取得とVMへの割り当て
@@ -87,21 +87,21 @@ GCE 仮想マシンは、ファイアウォールが存在するネットワー
{% data variables.product.prodname_ghe_server %} インスタンスを作成するには、{% data variables.product.prodname_ghe_server %} イメージを使用して GCE インスタンスを作成し、インスタンスデータ用の追加のストレージボリュームをアタッチする必要があります。 詳細は「[ハードウェアについて](#hardware-considerations)」を参照してください。
1. gcloud computeコマンドラインツールを使い、インスタンスデータのためのストレージボリュームとしてアタッチして使うデータディスクを作成し、そのサイズをユーザライセンス数に基づいて設定してください。 詳しい情報については、Google ドキュメンテーションの「[gcloud compute disks create](https://cloud.google.com/sdk/gcloud/reference/compute/disks/create)」を参照してください。
- ```shell
- $ gcloud compute disks create DATA-DISK-NAME --size DATA-DISK-SIZE --type DATA-DISK-TYPE --zone ZONE
- ```
+ ```shell
+ $ gcloud compute disks create DATA-DISK-NAME --size DATA-DISK-SIZE --type DATA-DISK-TYPE --zone ZONE
+ ```
2. 次に、選択した {% data variables.product.prodname_ghe_server %} イメージの名前を使用してインスタンスを作成し、データディスクをアタッチします。 詳しい情報については、Googleドキュメンテーションの「[gcloud compute instances create](https://cloud.google.com/sdk/gcloud/reference/compute/instances/create)」を参照してください。
- ```shell
- $ gcloud compute instances create INSTANCE-NAME \
- --machine-type n1-standard-8 \
- --image GITHUB-ENTERPRISE-IMAGE-NAME \
- --disk name=DATA-DISK-NAME \
- --metadata serial-port-enable=1 \
- --zone ZONE \
- --network NETWORK-NAME \
- --image-project github-enterprise-public
- ```
+ ```shell
+ $ gcloud compute instances create INSTANCE-NAME \
+ --machine-type n1-standard-8 \
+ --image GITHUB-ENTERPRISE-IMAGE-NAME \
+ --disk name=DATA-DISK-NAME \
+ --metadata serial-port-enable=1 \
+ --zone ZONE \
+ --network NETWORK-NAME \
+ --image-project github-enterprise-public
+ ```
### インスタンスの設定
diff --git a/translations/ja-JP/content/admin/overview/about-enterprise-accounts.md b/translations/ja-JP/content/admin/overview/about-enterprise-accounts.md
index a12519611f6e..7a6384cef929 100644
--- a/translations/ja-JP/content/admin/overview/about-enterprise-accounts.md
+++ b/translations/ja-JP/content/admin/overview/about-enterprise-accounts.md
@@ -1,26 +1,31 @@
---
title: Enterprise アカウントについて
-intro: '{% data variables.product.prodname_ghe_server %} を使用すると、Enterprise アカウントを作成して、管理者に支払いとライセンスの使用に関する単一の表示と管理ポイントを提供できます。'
+intro: 'With {% data variables.product.product_name %}, you can use an enterprise account to give administrators a single point of visibility and management{% if enterpriseServerVersions contains currentVersion %} for billing and license usage{% endif %}.'
redirect_from:
- /enterprise/admin/installation/about-enterprise-accounts
- /enterprise/admin/overview/about-enterprise-accounts
versions:
- enterprise-server: '*'
+ enterprise-server: '>=2.20'
+ github-ae: '*'
---
-### {% data variables.product.prodname_ghe_server %} の Enterprise アカウントについて
+### {% data variables.product.product_name %} の Enterprise アカウントについて
-Enterprise アカウントでは、複数の {% data variables.product.prodname_dotcom %} Organization と {% data variables.product.prodname_ghe_server %} インスタンスを管理できます。 Enterprise アカウントは、{% data variables.product.prodname_dotcom %} 上の Organization や個人アカウントのようにハンドルを持たなければなりません。 Enterprise 管理者は、以下のような設定やプリファレンスを管理できます:
+An enterprise account allows you to manage multiple organizations{% if enterpriseServerVersions contains currentVersion %} and {% data variables.product.prodname_ghe_server %} instances{% else %} on {% data variables.product.product_name %}{% endif %}. Enterprise アカウントは、{% data variables.product.prodname_dotcom %} 上の Organization や個人アカウントのようにハンドルを持たなければなりません。 Enterprise 管理者は、以下のような設定やプリファレンスを管理できます:
-- メンバーのアクセスと管理 (Organization のメンバー、外部コラボレーター)
-- 支払いと使用状況 ({% data variables.product.prodname_ghe_server %} インスタンス、ユーザライセンス、{% data variables.large_files.product_name_short %} パック)
-- セキュリティ (シングルサインオン、2 要素認証)
-- {% data variables.contact.enterprise_support %} とのリクエストおよび Support Bundle の共有
+- Member access and management (organization members, outside collaborators){% if enterpriseServerVersions contains currentVersion %}
+- Billing and usage ({% data variables.product.prodname_ghe_server %} instances, user licenses, {% data variables.large_files.product_name_short %} packs){% endif %}
+- Security{% if enterpriseServerVersions contains currentVersion %}(single sign-on, two factor authentication)
+- Requests {% if enterpriseServerVersions contains currentVersion %}and support bundle sharing {% endif %}with {% data variables.contact.enterprise_support %}{% endif %}
-{% data reusables.enterprise-accounts.enterprise-accounts-billing %}
+{% if enterpriseServerVersions contains currentVersion %}{% data reusables.enterprise-accounts.enterprise-accounts-billing %} For more information about managing your {% data variables.product.prodname_ghe_cloud %} subscription, see "[Viewing the subscription and usage for your enterprise account](/articles/viewing-the-subscription-and-usage-for-your-enterprise-account)." {% endif %}For more information about managing your {% data variables.product.product_name %} billing settings, see "[Managing billing for your enterprise](/admin/overview/managing-billing-for-your-enterprise)."
+
+{% if enterpriseServerVersions contains currentVersion %}
{% data variables.product.prodname_ghe_cloud %} と {% data variables.product.prodname_ghe_server %} の違いについては、「[{% data variables.product.prodname_dotcom %} の製品](/articles/githubs-products)」を参照してください。 {% data variables.product.prodname_enterprise %} にアップグレードする、または Enterprise アカウントを使い始める場合は、{% data variables.contact.contact_enterprise_sales %} にお問い合わせください。
### Enterprise アカウントにリンクされている {% data variables.product.prodname_ghe_server %} ライセンスの管理
{% data reusables.enterprise-accounts.admin-managing-licenses %}
+
+{% endif %}
diff --git a/translations/ja-JP/content/admin/packages/configuring-third-party-storage-for-packages.md b/translations/ja-JP/content/admin/packages/configuring-third-party-storage-for-packages.md
index baa3a136f49e..cae7bc8a653d 100644
--- a/translations/ja-JP/content/admin/packages/configuring-third-party-storage-for-packages.md
+++ b/translations/ja-JP/content/admin/packages/configuring-third-party-storage-for-packages.md
@@ -13,7 +13,7 @@ versions:
{% data variables.product.prodname_ghe_server %} 上の {% data variables.product.prodname_registry %} は、外部の blob ストレージを使用してパッケージを保存します。 必要なストレージ容量は、{% data variables.product.prodname_registry %} の使用状況によって異なります。
-現時点では、{% data variables.product.prodname_registry %} は Amazon Web Services (AWS) S3 で blob ストレージをサポートしています。 MinIO もサポートされていますが、設定は現在 {% data variables.product.product_name %} インタフェースに実装されていません。 You can use MinIO for storage by following the instructions for AWS S3, entering the analogous information for your MinIO configuration.
+現時点では、{% data variables.product.prodname_registry %} は Amazon Web Services (AWS) S3 で blob ストレージをサポートしています。 MinIO もサポートされていますが、設定は現在 {% data variables.product.product_name %} インタフェースに実装されていません。 You can use MinIO for storage by following the instructions for AWS S3, entering the analogous information for your MinIO configuration. Before configuring third-party storage for {% data variables.product.prodname_registry %} on {% data variables.product.prodname_dotcom %}, you must set up a bucket with your third-party storage provider. For more information on installing and running a MinIO bucket to use with {% data variables.product.prodname_registry %}, see the "[Quickstart for configuring MinIO storage](/admin/packages/quickstart-for-configuring-minio-storage)."
最適なエクスペリエンスを得るには、{% data variables.product.prodname_actions %} のストレージに使用するバケットとは別に、{% data variables.product.prodname_registry %} }専用のバケットを使用することをお勧めします。
diff --git a/translations/ja-JP/content/admin/packages/index.md b/translations/ja-JP/content/admin/packages/index.md
index b3060eb8b17b..3ca9c8e5751f 100644
--- a/translations/ja-JP/content/admin/packages/index.md
+++ b/translations/ja-JP/content/admin/packages/index.md
@@ -10,5 +10,6 @@ versions:
{% data reusables.package_registry.packages-ghes-release-stage %}
{% link_with_intro /enabling-github-packages-for-your-enterprise %}
+{% link_with_intro /quickstart-for-configuring-minio-storage %}
{% link_with_intro /configuring-packages-support-for-your-enterprise %}
{% link_with_intro /configuring-third-party-storage-for-packages %}
diff --git a/translations/ja-JP/content/admin/packages/quickstart-for-configuring-minio-storage.md b/translations/ja-JP/content/admin/packages/quickstart-for-configuring-minio-storage.md
new file mode 100644
index 000000000000..415eda723dc4
--- /dev/null
+++ b/translations/ja-JP/content/admin/packages/quickstart-for-configuring-minio-storage.md
@@ -0,0 +1,133 @@
+---
+title: Quickstart for configuring MinIO storage
+intro: 'Set up MinIO as a storage provider for using {% data variables.product.prodname_registry %} on your enterprise.'
+versions:
+ enterprise-server: '>=2.22'
+---
+
+{% data reusables.package_registry.packages-ghes-release-stage %}
+
+Before you can enable and configure {% data variables.product.prodname_registry %} on {% data variables.product.product_location_enterprise %}, you need to prepare your third-party storage solution.
+
+MinIO offers object storage with support for the S3 API and {% data variables.product.prodname_registry %} on your enterprise.
+
+This quickstart shows you how to set up MinIO using Docker for use with {% data variables.product.prodname_registry %} but you have other options for managing MinIO besides Docker. For more information about MinIO, see the official [MinIO docs](https://docs.min.io/).
+
+### 1. Choose a MinIO mode for your needs
+
+| MinIO mode | Optimized for | Storage infrastructure required |
+| ----------------------------------------------- | ------------------------------ | ------------------------------------ |
+| Standalone MinIO (on a single host) | Fast setup | なし |
+| MinIO as a NAS gateway | NAS (Network-attached storage) | NAS devices |
+| Clustered MinIO (also called Distributed MinIO) | Data security | Storage servers running in a cluster |
+
+For more information about your options, see the official [MinIO docs](https://docs.min.io/).
+
+### 2. Install, run, and sign in to MinIO
+
+1. Set up your preferred environment variables for MinIO.
+
+ These examples use `MINIO_DIR`:
+ ```shell
+ $ export MINIO_DIR=$(pwd)/minio
+ $ mkdir -p $MINIO_DIR
+ ```
+
+2. Install MinIO.
+
+ ```shell
+ $ docker pull minio/minio
+ ```
+ For more information, see the official "[MinIO Quickstart Guide](https://docs.min.io/docs/minio-quickstart-guide)."
+
+3. Sign in to MinIO using your MinIO access key and secret.
+
+ {% linux %}
+ ```shell
+ $ export MINIO_ACCESS_KEY=$(cat /dev/urandom | tr -dc 'a-zA-Z0-9' | fold -w 32 | head -n 1)
+ # this one is actually a secret, so careful
+ $ export MINIO_SECRET_KEY=$(cat /dev/urandom | tr -dc 'a-zA-Z0-9' | fold -w 32 | head -n 1)
+ ```
+ {% endlinux %}
+
+ {% mac %}
+ ```shell
+ $ export MINIO_ACCESS_KEY=$(cat /dev/urandom | LC_CTYPE=C tr -dc 'a-zA-Z0-9' | fold -w 32 | head -n 1)
+ # this one is actually a secret, so careful
+ $ export MINIO_SECRET_KEY=$(cat /dev/urandom | LC_CTYPE=C tr -dc 'a-zA-Z0-9' | fold -w 32 | head -n 1)
+ ```
+ {% endmac %}
+
+ You can access your MinIO keys using the environment variables:
+
+ ```shell
+ $ echo $MINIO_ACCESS_KEY
+ $ echo $MINIO_SECRET_KEY
+ ```
+
+4. Run MinIO in your chosen mode.
+
+ * Run MinIO using Docker on a single host:
+
+ ```shell
+ $ docker run -p 9000:9000 \
+ -v $MINIO_DIR:/data \
+ -e "MINIO_ACCESS_KEY=$MINIO_ACCESS_KEY" \
+ -e "MINIO_SECRET_KEY=$MINIO_SECRET_KEY" \
+ minio/minio server /data
+ ```
+
+ For more information, see "[MinIO Docker Quickstart guide](https://docs.min.io/docs/minio-docker-quickstart-guide.html)."
+
+ * Run MinIO using Docker as a NAS gateway:
+
+ This setup is useful for deployments where there is already a NAS you want to use as the backup storage for {% data variables.product.prodname_registry %}.
+
+ ```shell
+ $ docker run -p 9000:9000 \
+ -v $MINIO_DIR:/data \
+ -e "MINIO_ACCESS_KEY=$MINIO_ACCESS_KEY" \
+ -e "MINIO_SECRET_KEY=$MINIO_SECRET_KEY" \
+ minio/minio gateway nas /data
+ ```
+
+ For more information, see "[MinIO Gateway for NAS](https://docs.min.io/docs/minio-gateway-for-nas.html)."
+
+ * Run MinIO using Docker as a cluster. This MinIO deployment uses several hosts and MinIO's erasure coding for the strongest data protection. To run MinIO in a cluster mode, see the "[Distributed MinIO Quickstart Guide](https://docs.min.io/docs/distributed-minio-quickstart-guide.html).
+
+### 3. Create your MinIO bucket for {% data variables.product.prodname_registry %}
+
+1. Install the MinIO client.
+
+ ```shell
+ $ docker pull minio/mc
+ ```
+
+2. Create a bucket with a host URL that {% data variables.product.prodname_ghe_server %} can access.
+
+ * Local deployments example:
+
+ ```shell
+ $ export MC_HOST_minio="http://${MINIO_ACCESS_KEY}:${MINIO_SECRET_KEY} @localhost:9000"
+ $ docker run minio/mc BUCKET-NAME
+ ```
+
+ This example can be used for MinIO standalone or MinIO as a NAS gateway.
+
+ * Clustered deployments example:
+
+ ```shell
+ $ export MC_HOST_minio="http://${MINIO_ACCESS_KEY}:${MINIO_SECRET_KEY} @minioclustername.example.com:9000"
+ $ docker run minio/mc mb packages
+ ```
+
+
+### 次のステップ
+
+To finish configuring storage for {% data variables.product.prodname_registry %}, you'll need to copy the MinIO storage URL:
+
+ ```
+ echo "http://${MINIO_ACCESS_KEY}:${MINIO_SECRET_KEY}@minioclustername.example.com:9000"
+ ```
+
+For the next steps, see "[Configuring third-party storage for packages](/admin/packages/configuring-third-party-storage-for-packages)."
\ No newline at end of file
diff --git a/translations/ja-JP/content/admin/policies/creating-a-pre-receive-hook-environment.md b/translations/ja-JP/content/admin/policies/creating-a-pre-receive-hook-environment.md
index 52e1bef71d96..94b580ea6f95 100644
--- a/translations/ja-JP/content/admin/policies/creating-a-pre-receive-hook-environment.md
+++ b/translations/ja-JP/content/admin/policies/creating-a-pre-receive-hook-environment.md
@@ -21,10 +21,10 @@ pre-receiveフック環境の構築には、Linuxのコンテナ管理ツール
{% data reusables.linux.ensure-docker %}
2. この情報を含む `Dockerfile.alpine-3.3` ファイルを作成してください:
- ```
- FROM gliderlabs/alpine:3.3
- RUN apk add --no-cache git bash
- ```
+ ```
+ FROM gliderlabs/alpine:3.3
+ RUN apk add --no-cache git bash
+ ```
3. `Dockerfile.alpine-3.3`を含むワーキングディレクトリから、イメージをビルドします:
```shell
@@ -36,37 +36,37 @@ pre-receiveフック環境の構築には、Linuxのコンテナ管理ツール
> ---> Using cache
> ---> 0250ab3be9c5
> Successfully built 0250ab3be9c5
- ```
+ ```
4. コンテナを作成します:
```shell
$ docker create --name pre-receive.alpine-3.3 pre-receive.alpine-3.3 /bin/true
- ```
+ ```
5. この Docker コンテナを `gzip` 圧縮された `tar` ファイルにエクスポートします:
```shell
$ docker export pre-receive.alpine-3.3 | gzip > alpine-3.3.tar.gz
- ```
+ ```
- このファイル `alpine-3.3.tar.gz` を {% data variables.product.prodname_ghe_server %} アプライアンスにアップロードする準備ができました。
+ このファイル `alpine-3.3.tar.gz` を {% data variables.product.prodname_ghe_server %} アプライアンスにアップロードする準備ができました。
### chrootを使ったpre-receiveフック環境の作成
1. Linux の `chroot` 環境を作成します。
2. `chroot` ディレクトリの `gzip` 圧縮された `tar` ファイルを作成します.
- ```shell
- $ cd /path/to/chroot
- $ tar -czf /path/to/pre-receive-environment.tar.gz .
+ ```shell
+ $ cd /path/to/chroot
+ $ tar -czf /path/to/pre-receive-environment.tar.gz .
```
- {% note %}
+ {% note %}
- **ノート:**
- - `/path/to/chroot`のような、ファイルの先行するディレクトリパスをtarアーカイブに含めないでください。
- - chroot環境へのエントリポイントとして、`/bin/sh`が存在し、実行可能でなければなりません。
- - 旧来のchrootと異なり、`dev`ディレクトリはpre-receiveフックのためのchroot環境では必要ありません。
+ **ノート:**
+ - `/path/to/chroot`のような、ファイルの先行するディレクトリパスをtarアーカイブに含めないでください。
+ - chroot環境へのエントリポイントとして、`/bin/sh`が存在し、実行可能でなければなりません。
+ - 旧来のchrootと異なり、`dev`ディレクトリはpre-receiveフックのためのchroot環境では必要ありません。
- {% endnote %}
+ {% endnote %}
chroot 環境の作成に関する詳しい情報については *Debian Wiki* の「[Chroot](https://wiki.debian.org/chroot)」、*Ubuntu Community Help Wiki* の「[BasicChroot](https://help.ubuntu.com/community/BasicChroot)」、または *Alpine Linux Wiki* の「[Installing Alpine Linux in a chroot](http://wiki.alpinelinux.org/wiki/Installing_Alpine_Linux_in_a_chroot)」を参照してください。
@@ -89,4 +89,4 @@ chroot 環境の作成に関する詳しい情報については *Debian Wiki*
```shell
admin@ghe-host:~$ ghe-hook-env-create AlpineTestEnv /home/admin/alpine-3.3.tar.gz
> Pre-receive hook environment 'AlpineTestEnv' (2) has been created.
- ```
+ ```
diff --git a/translations/ja-JP/content/admin/policies/creating-a-pre-receive-hook-script.md b/translations/ja-JP/content/admin/policies/creating-a-pre-receive-hook-script.md
index 37c3f79ffc23..b9ef095954d7 100644
--- a/translations/ja-JP/content/admin/policies/creating-a-pre-receive-hook-script.md
+++ b/translations/ja-JP/content/admin/policies/creating-a-pre-receive-hook-script.md
@@ -70,19 +70,19 @@ pre-receive フックスクリプトは、{% data variables.product.prodname_ghe
```shell
$ sudo chmod +x SCRIPT_FILE.sh
- ```
- Windows ユーザは、スクリプトに実行権限を持たせてください。
+ ```
+ Windows ユーザは、スクリプトに実行権限を持たせてください。
- ```shell
- git update-index --chmod=+x SCRIPT_FILE.sh
- ```
+ ```shell
+ git update-index --chmod=+x SCRIPT_FILE.sh
+ ```
2. {% data variables.product.prodname_ghe_server %} インスタンス上の対象となる pre-receive フックのリポジトリにコミットおよびプッシュしてください。
```shell
$ git commit -m "YOUR COMMIT MESSAGE"
$ git push
- ```
+ ```
3. {% data variables.product.prodname_ghe_server %} のインスタンス上で [pre-receive フックを作成](/enterprise/{{ currentVersion }}/admin/guides/developer-workflow/managing-pre-receive-hooks-on-the-github-enterprise-appliance/#creating-pre-receive-hooks)してください。
@@ -93,40 +93,40 @@ pre-receive フックスクリプトは、{% data variables.product.prodname_ghe
2. 以下を含む `Dockerfile.dev` というファイルを作成してください。
- ```
- FROM gliderlabs/alpine:3.3
- RUN \
- apk add --no-cache git openssh bash && \
- ssh-keygen -A && \
- sed -i "s/#AuthorizedKeysFile/AuthorizedKeysFile/g" /etc/ssh/sshd_config && \
- adduser git -D -G root -h /home/git -s /bin/bash && \
- passwd -d git && \
- su git -c "mkdir /home/git/.ssh && \
- ssh-keygen -t ed25519 -f /home/git/.ssh/id_ed25519 -P '' && \
- mv /home/git/.ssh/id_ed25519.pub /home/git/.ssh/authorized_keys && \
- mkdir /home/git/test.git && \
- git --bare init /home/git/test.git"
-
- VOLUME ["/home/git/.ssh", "/home/git/test.git/hooks"]
- WORKDIR /home/git
-
- CMD ["/usr/sbin/sshd", "-D"]
- ```
+ ```
+ FROM gliderlabs/alpine:3.3
+ RUN \
+ apk add --no-cache git openssh bash && \
+ ssh-keygen -A && \
+ sed -i "s/#AuthorizedKeysFile/AuthorizedKeysFile/g" /etc/ssh/sshd_config && \
+ adduser git -D -G root -h /home/git -s /bin/bash && \
+ passwd -d git && \
+ su git -c "mkdir /home/git/.ssh && \
+ ssh-keygen -t ed25519 -f /home/git/.ssh/id_ed25519 -P '' && \
+ mv /home/git/.ssh/id_ed25519.pub /home/git/.ssh/authorized_keys && \
+ mkdir /home/git/test.git && \
+ git --bare init /home/git/test.git"
+
+ VOLUME ["/home/git/.ssh", "/home/git/test.git/hooks"]
+ WORKDIR /home/git
+
+ CMD ["/usr/sbin/sshd", "-D"]
+ ```
3. `always_reject.sh` というテストのpre-receiveスクリプトを作成してください。 このスクリプト例では、全てのプッシュを拒否します。これは、リポジトリをロックする場合に役立ちます。
- ```
- #!/usr/bin/env bash
+ ```
+ #!/usr/bin/env bash
- echo "error: rejecting all pushes"
- exit 1
- ```
+ echo "error: rejecting all pushes"
+ exit 1
+ ```
4. `always_reject.sh`スクリプトが実行権限を持つことを確認してください。
```shell
$ chmod +x always_reject.sh
- ```
+ ```
5. `Dockerfile.dev` を含むディレクトリからイメージをビルドしてください。
@@ -149,32 +149,32 @@ pre-receive フックスクリプトは、{% data variables.product.prodname_ghe
....出力を省略....
> Initialized empty Git repository in /home/git/test.git/
> Successfully built dd8610c24f82
- ```
+ ```
6. 生成された SSH キーを含むデータコンテナを実行してください。
```shell
$ docker run --name data pre-receive.dev /bin/true
- ```
+ ```
7. テスト pre-receive フックの `always_reject.sh` をデータコンテナにコピーしてください:
```shell
$ docker cp always_reject.sh data:/home/git/test.git/hooks/pre-receive
- ```
+ ```
8. `sshd` を実行しフックを動作させるアプリケーションコンテナを実行してください。 返されたコンテナ ID をメモしておいてください:
```shell
$ docker run -d -p 52311:22 --volumes-from data pre-receive.dev
> 7f888bc700b8d23405dbcaf039e6c71d486793cad7d8ae4dd184f4a47000bc58
- ```
+ ```
9. 生成された SSH キーをデータコンテナからローカルマシンにコピーしてください:
```shell
$ docker cp data:/home/git/.ssh/id_ed25519 .
- ```
+ ```
10. テストリポジトリのリモートを修正して、Docker コンテナ内の `test.git` リポジトリにプッシュしてください。 この例では `git@github.com:octocat/Hello-World.git` を使っていますが、どのリポジトリを使ってもかまいません。 この例ではローカルマシン (127.0.0.1) がポート 52311 をバインドしているものとしていますが、docker がリモートマシンで動作しているなら異なる IP アドレスを使うことができます。
@@ -193,9 +193,9 @@ pre-receive フックスクリプトは、{% data variables.product.prodname_ghe
> To git@192.168.99.100:test.git
> ! [remote rejected] main -> main (pre-receive hook declined)
> error: failed to push some refs to 'git@192.168.99.100:test.git'
- ```
+ ```
- pre-receive フックの実行後にプッシュが拒否され、スクリプトからの出力がエコーされていることに注意してください。
+ pre-receive フックの実行後にプッシュが拒否され、スクリプトからの出力がエコーされていることに注意してください。
### 参考リンク
- *Pro Git Webサイト*の「[Gitのカスタマイズ - Gitポリシーの実施例](https://git-scm.com/book/en/v2/Customizing-Git-An-Example-Git-Enforced-Policy)」
diff --git a/translations/ja-JP/content/admin/policies/enforcing-repository-management-policies-in-your-enterprise.md b/translations/ja-JP/content/admin/policies/enforcing-repository-management-policies-in-your-enterprise.md
index dd8be775cb4d..5885c0a28f83 100644
--- a/translations/ja-JP/content/admin/policies/enforcing-repository-management-policies-in-your-enterprise.md
+++ b/translations/ja-JP/content/admin/policies/enforcing-repository-management-policies-in-your-enterprise.md
@@ -34,7 +34,7 @@ versions:
Each time someone creates a new repository on your enterprise, that person must choose a visibility for the repository. When you configure a default visibility setting for the enterprise, you choose which visibility is selected by default. リポジトリの可視性に関する詳しい情報については、「[リポジトリの可視性について](/github/creating-cloning-and-archiving-repositories/about-repository-visibility)」を参照してください。
-サイト管理者がメンバーに対して特定の種類のリポジトリの作成を禁止している場合、可視性の設定のデフォルトがその種類になっていても、メンバーはその種類のリポジトリを作成できません。 For more information, see "[Setting a policy for repository creation](#setting-a-policy-for-repository-creation)."
+If an enterprise owner disallows members from creating certain types of repositories, members will not be able to create that type of repository even if the visibility setting defaults to that type. For more information, see "[Setting a policy for repository creation](#setting-a-policy-for-repository-creation)."
{% data reusables.enterprise-accounts.access-enterprise %}
{% if currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
@@ -49,9 +49,9 @@ Each time someone creates a new repository on your enterprise, that person must
### リポジトリの可視性を変更するためのポリシーを設定する
-メンバーがリポジトリの可視性を変更できないようにすると、パブリックなリポジトリをプライベートにしたり、プライベートなリポジトリをパブリックにしたりできるのはサイト管理者だけになります。
+When you prevent members from changing repository visibility, only enterprise owners can change the visibility of a repository.
-サイト管理者がリポジトリの作成を Organization のオーナーのみに制限している場合、メンバーはリポジトリの可視性を変更できません。 サイト管理者がメンバーのリポジトリ作成をプライベート リポジトリのみに制限している場合、メンバーはリポジトリをパブリックからプライベートに変更すること以外はできません。 For more information, see "[Setting a policy for repository creation](#setting-a-policy-for-repository-creation)."
+If an enterprise owner has restricted repository creation to organization owners only, then members will not be able to change repository visibility. If an enterprise owner has restricted member repository creation to private repositories only, then members will only be able to change the visibility of a repository to private. For more information, see "[Setting a policy for repository creation](#setting-a-policy-for-repository-creation)."
{% data reusables.enterprise-accounts.access-enterprise %}
{% data reusables.enterprise-accounts.policies-tab %}
@@ -75,6 +75,15 @@ Each time someone creates a new repository on your enterprise, that person must
6. [Repository creation(リポジトリの作成)] で、ドロップダウンメニューを使用してポリシーを選択します。 
{% endif %}
+### プライベートまたは内部リポジトリのフォークに関するポリシーを施行する
+
+Across all organizations owned by your enterprise, you can allow people with access to a private or internal repository to fork the repository, never allow forking of private or internal repositories, or allow owners to administer the setting on the organization level.
+
+{% data reusables.enterprise-accounts.access-enterprise %}
+{% data reusables.enterprise-accounts.policies-tab %}
+3. [**Repository policies**] タブの [Repository forking] で、設定変更についての情報を読みます。 {% data reusables.enterprise-accounts.view-current-policy-config-orgs %}
+4. [Repository forking] で、ドロップダウンメニューを使用してポリシーを選択します。 
+
### リポジトリの削除と移譲のためのポリシーを設定する
{% data reusables.enterprise-accounts.access-enterprise %}
@@ -166,6 +175,8 @@ To keep your repository size manageable and prevent performance issues, you can
- [**Block to the default branch(デフォルトブランチへのブロック)**] でデフォルトブランチへのフォースプッシュのみがブロックされます。 
6. **Enforce on all repositories(すべてのリポジトリに対して強制)**を選択して、リポジトリ固有の設定を上書きすることもできます。 Note that this will **not** override an enterprise-wide policy. 
+{% if enterpriseServerVersions contains currentVersion %}
+
### 匿名 Git 読み取りアクセスを設定する
{% data reusables.enterprise_user_management.disclaimer-for-git-read-access %}
@@ -192,7 +203,6 @@ If necessary, you can prevent repository administrators from changing anonymous
4. [Anonymous Git read access(匿名 Git 読み取りアクセス)] の下で、ドロップダウンメニューを使って [**Enabled(有効化)**] をクリックしてください。 ![[Enabled] と [Disabled] のメニューオプションが表示されている [Anonymous Git read access] ドロップダウンメニュー](/assets/images/enterprise/site-admin-settings/enable-anonymous-git-read-access.png)
3. Optionally, to prevent repository admins from changing anonymous Git read access settings in all repositories on your enterprise, select **Prevent repository admins from changing anonymous Git read access**. 
-{% if enterpriseServerVersions contains currentVersion %}
#### 特定のリポジトリでの匿名 Git 読み取りアクセスを設定する
{% data reusables.enterprise_site_admin_settings.access-settings %}
@@ -203,6 +213,7 @@ If necessary, you can prevent repository administrators from changing anonymous
6. "Danger Zone(危険区域)"の下で、"Enable Anonymous Git read access(匿名Git読み取りアクセスの有効化)"の隣の**Enable(有効化)**をクリックしてください。 
7. 変更を確認します。 確定するには、[**Yes, enable anonymous Git read access**] をクリックします。 ![ポップアップウィンドウの [Confirm anonymous Git read access] 設定](/assets/images/enterprise/site-admin-settings/confirm-anonymous-git-read-access-for-specific-repo-as-site-admin.png)
8. このリポジトリの設定をリポジトリ管理者が変更するのを避けるために、[**Prevent repository admins from changing anonymous Git read access(リポジトリ管理者による匿名Git読み取りアクセスの変更の回避)**] を選択することもできます。 
+
{% endif %}
{% if currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
diff --git a/translations/ja-JP/content/admin/release-notes.md b/translations/ja-JP/content/admin/release-notes.md
new file mode 100644
index 000000000000..4fedc31ab998
--- /dev/null
+++ b/translations/ja-JP/content/admin/release-notes.md
@@ -0,0 +1,8 @@
+---
+title: リリースノート
+intro: The release notes for {{ allVersions[currentVersion].versionTitle }}.
+layout: release-notes
+versions:
+ enterprise-server: '*'
+---
+
diff --git a/translations/ja-JP/content/admin/user-management/audited-actions.md b/translations/ja-JP/content/admin/user-management/audited-actions.md
index 44f6df7aa5a5..4de3710f1afa 100644
--- a/translations/ja-JP/content/admin/user-management/audited-actions.md
+++ b/translations/ja-JP/content/admin/user-management/audited-actions.md
@@ -36,12 +36,12 @@ versions:
#### Enterprise configuration settings
-| 名前 | 説明 |
-| -------------------------------------------------------:| ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `business.update_member_repository_creation_permission` | A site admin restricts repository creation in organizations in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#setting-a-policy-for-repository-creation)." |
-| `business.clear_members_can_create_repos` | A site admin clears a restriction on repository creation in organizations in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#setting-a-policy-for-repository-creation)." |
-| `enterprise.config.lock_anonymous_git_access` | A site admin locks anonymous Git read access to prevent repository admins from changing existing anonymous Git read access settings for repositories in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#configuring-anonymous-git-read-access)." |
-| `enterprise.config.unlock_anonymous_git_access` | A site admin unlocks anonymous Git read access to allow repository admins to change existing anonymous Git read access settings for repositories in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#configuring-anonymous-git-read-access)." |
+| 名前 | 説明 |
+| -------------------------------------------------------:| -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `business.update_member_repository_creation_permission` | A site admin restricts repository creation in organizations in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#setting-a-policy-for-repository-creation)." |
+| `business.clear_members_can_create_repos` | A site admin clears a restriction on repository creation in organizations in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#setting-a-policy-for-repository-creation)."{% if enterpriseServerVersions contains currentVersion %}
+| `enterprise.config.lock_anonymous_git_access` | A site admin locks anonymous Git read access to prevent repository admins from changing existing anonymous Git read access settings for repositories in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#configuring-anonymous-git-read-access)." |
+| `enterprise.config.unlock_anonymous_git_access` | A site admin unlocks anonymous Git read access to allow repository admins to change existing anonymous Git read access settings for repositories in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#configuring-anonymous-git-read-access)."{% endif %}
#### Issue およびプルリクエスト
@@ -77,23 +77,23 @@ versions:
#### リポジトリ
-| 名前 | 説明 |
-| ------------------------------------------:| ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `repo.access` | プライベートリポジトリが公開されたか、パブリックリポジトリが非公開にされました。 |
-| `repo.archive` | リポジトリがアーカイブされました。 For more information, see "[Archiving a {% data variables.product.prodname_dotcom %} repository](/github/creating-cloning-and-archiving-repositories/archiving-a-github-repository)." |
-| `repo.add_member` | リポジトリにコラボレーターが追加されました。 |
-| `repo.config` | サイト管理者がフォースプッシュをブロックしました。 詳しくは、 [リポジトリへのフォースプッシュのブロック](/enterprise/{{ currentVersion }}/admin/guides/developer-workflow/blocking-force-pushes-to-a-repository/)を参照してください。 |
-| `repo.create` | リポジトリが作成されました。 |
-| `repo.destroy` | リポジトリが削除されました。 |
-| `repo.remove_member` | コラボレーターがリポジトリから削除されました。 |
-| `repo.rename` | リポジトリの名前が変更されました。 |
-| `repo.transfer` | ユーザーが転送されたリポジトリを受け取る要求を受け入れました。 |
-| `repo.transfer_start` | ユーザーがリポジトリを別のユーザーまたは Organization に転送する要求を送信しました。 |
-| `repo.unarchive` | リポジトリがアーカイブ解除されました。 For more information, see "[Archiving a {% data variables.product.prodname_dotcom %} repository](/github/creating-cloning-and-archiving-repositories/archiving-a-github-repository)." |
-| `repo.config.disable_anonymous_git_access` | 匿名 Git 読み取りアクセスがパブリックリポジトリに対して無効になります。 詳細は「[リポジトリに対する匿名 Git 読み取りアクセスを有効化する](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)」を参照してください。 |
-| `repo.config.enable_anonymous_git_access` | 匿名 Git 読み取りアクセスがパブリックリポジトリに対して有効になっています。 詳細は「[リポジトリに対する匿名 Git 読み取りアクセスを有効化する](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)」を参照してください。 |
-| `repo.config.lock_anonymous_git_access` | リポジトリの匿名 Git 読み取りアクセス設定がロックされているため、リポジトリ管理者はこの設定を変更 (有効化または無効化) できません。 詳細は「[ユーザによる匿名 Git 読み取りアクセスの変更を禁止する](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)」を参照してください。 |
-| `repo.config.unlock_anonymous_git_access` | リポジトリの匿名 Git 読み取りアクセス設定がロック解除されているため、リポジトリ管理者はこの設定を変更 (有効化または無効化) できます。 詳細は「[ユーザによる匿名 Git 読み取りアクセスの変更を禁止する](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)」を参照してください。 |
+| 名前 | 説明 |
+| ------------------------------------------:| -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `repo.access` | The visibility of a repository changed to private{% if enterpriseServerVersions contains currentVersion %}, public,{% endif %} or internal. |
+| `repo.archive` | リポジトリがアーカイブされました。 For more information, see "[Archiving a {% data variables.product.prodname_dotcom %} repository](/github/creating-cloning-and-archiving-repositories/archiving-a-github-repository)." |
+| `repo.add_member` | リポジトリにコラボレーターが追加されました。 |
+| `repo.config` | サイト管理者がフォースプッシュをブロックしました。 詳しくは、 [リポジトリへのフォースプッシュのブロック](/enterprise/{{ currentVersion }}/admin/guides/developer-workflow/blocking-force-pushes-to-a-repository/)を参照してください。 |
+| `repo.create` | リポジトリが作成されました。 |
+| `repo.destroy` | リポジトリが削除されました。 |
+| `repo.remove_member` | コラボレーターがリポジトリから削除されました。 |
+| `repo.rename` | リポジトリの名前が変更されました。 |
+| `repo.transfer` | ユーザーが転送されたリポジトリを受け取る要求を受け入れました。 |
+| `repo.transfer_start` | ユーザーがリポジトリを別のユーザーまたは Organization に転送する要求を送信しました。 |
+| `repo.unarchive` | リポジトリがアーカイブ解除されました。 For more information, see "[Archiving a {% data variables.product.prodname_dotcom %} repository](/github/creating-cloning-and-archiving-repositories/archiving-a-github-repository)."{% if enterpriseServerVersions contains currentVersion %}
+| `repo.config.disable_anonymous_git_access` | Anonymous Git read access is disabled for a repository. 詳細は「[リポジトリに対する匿名 Git 読み取りアクセスを有効化する](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)」を参照してください。 |
+| `repo.config.enable_anonymous_git_access` | Anonymous Git read access is enabled for a repository. 詳細は「[リポジトリに対する匿名 Git 読み取りアクセスを有効化する](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)」を参照してください。 |
+| `repo.config.lock_anonymous_git_access` | リポジトリの匿名 Git 読み取りアクセス設定がロックされているため、リポジトリ管理者はこの設定を変更 (有効化または無効化) できません。 詳細は「[ユーザによる匿名 Git 読み取りアクセスの変更を禁止する](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)」を参照してください。 |
+| `repo.config.unlock_anonymous_git_access` | リポジトリの匿名 Git 読み取りアクセス設定がロック解除されているため、リポジトリ管理者はこの設定を変更 (有効化または無効化) できます。 For more information, see "[Preventing users from changing anonymous Git read access](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)."{% endif %}
#### サイトアドミンのツール
diff --git a/translations/ja-JP/content/admin/user-management/managing-organizations-in-your-enterprise.md b/translations/ja-JP/content/admin/user-management/managing-organizations-in-your-enterprise.md
index 55ffb3383150..ac79e72f25ce 100644
--- a/translations/ja-JP/content/admin/user-management/managing-organizations-in-your-enterprise.md
+++ b/translations/ja-JP/content/admin/user-management/managing-organizations-in-your-enterprise.md
@@ -5,7 +5,7 @@ redirect_from:
- /enterprise/admin/categories/admin-bootcamp/
- /enterprise/admin/user-management/organizations-and-teams
- /enterprise/admin/user-management/managing-organizations-in-your-enterprise
-intro: 'Organizationは企業内で、部署や同様のプロジェクトで作業を行うグループなど、個別のユーザグループを作成する素晴らしい手段です。 Organizationに属するパブリックリポジトリは他のOrganizationのユーザからもアクセスできますが、プライベートリポジトリはOrganizationのメンバーでなければアクセスできません。'
+intro: 'Organizationは企業内で、部署や同様のプロジェクトで作業を行うグループなど、個別のユーザグループを作成する素晴らしい手段です。 {% if currentVersion == "github-ae@latest" %}Internal{% else %}Public and internal{% endif %} repositories that belong to an organization are accessible to users in other organizations, while private repositories are inaccessible to anyone but members of the organization that are granted access.'
mapTopic: true
versions:
enterprise-server: '*'
diff --git a/translations/ja-JP/content/developers/apps/authorizing-oauth-apps.md b/translations/ja-JP/content/developers/apps/authorizing-oauth-apps.md
index 3189fba3c23f..a6447d2aac9f 100644
--- a/translations/ja-JP/content/developers/apps/authorizing-oauth-apps.md
+++ b/translations/ja-JP/content/developers/apps/authorizing-oauth-apps.md
@@ -114,11 +114,13 @@ curl -H "Authorization: token OAUTH-TOKEN" {% data variables.product.api_url_pre
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
### デバイスフロー
+{% if currentVersion ver_lt "enterprise-server@3.1" %}
{% note %}
-**ノート:** デバイスフローはパブリックベータであり、変更されることがあります。{% if currentVersion == "free-pro-team@latest" %} このベータの機能を有効化するには、「[アプリケーションのベータ機能のアクティベート](/developers/apps/activating-beta-features-for-apps)」を参照してください。{% endif %}
+**Note:** The device flow is in public beta and subject to change.
{% endnote %}
+{% endif %}
デバイスフローを使えば、CLIツールやGit認証情報マネージャーなどのヘッドレスアプリケーションのユーザを認可できます。
diff --git a/translations/ja-JP/content/developers/apps/creating-ci-tests-with-the-checks-api.md b/translations/ja-JP/content/developers/apps/creating-ci-tests-with-the-checks-api.md
index fde1e38f58e4..6a9386ff9467 100644
--- a/translations/ja-JP/content/developers/apps/creating-ci-tests-with-the-checks-api.md
+++ b/translations/ja-JP/content/developers/apps/creating-ci-tests-with-the-checks-api.md
@@ -32,13 +32,13 @@ Checks API は、新しいコードがリポジトリにプッシュされるた
1. アプリケーションがこのイベントを受信すると、アプリケーションはスイートに[チェック実行を追加](/v3/checks/runs/#create-a-check-run)できます。
1. チェック実行には、コードの特定の行で表示される[アノテーション](/v3/checks/runs/#annotations-object)を含めることができます。
-**In this guide, you’ll learn how to:**
+**このガイドでは、次のこと行う方法について学びます。**
* パート 1: Checks API を使用して CI サーバー用のフレームワークをセットアップする。
* Checks API イベントを受信するサーバーとして GitHub App を構成します。
* Create new check runs for CI tests when a repository receives newly pushed commits.
* Re-run check runs when a user requests that action on GitHub.
-* Part 2: Build on the CI server framework you created by adding a linter CI test.
+* パート 2: 文法チェッカー CI テストを追加して、作成した CI サーバーフレームワークを基に構築する。
* Update a check run with a `status`, `conclusion`, and `output` details.
* Create annotations on lines of code that GitHub displays in the **Checks** and **Files Changed** tab of a pull request.
* Automatically fix linter recommendations by exposing a "Fix this" button in the **Checks** tab of the pull request.
@@ -51,7 +51,7 @@ Checks API は、新しいコードがリポジトリにプッシュされるた
以下の作業に取りかかる前に、[Github Apps](/apps/)、[webhook](/webhooks)、[Checks API](/v3/checks/) を使い慣れていない場合は、ある程度慣れておくとよいでしょう。 [REST API ドキュメント](/v3/)には、さらに API が掲載されています。 Checks API は [GraphQL](/v4/) でも使用できますが、このクイックスタートでは REST に焦点を当てます。 詳細については、GraphQL [Checks Suite](/v4/object/checksuite/) および [Check Run](/v4/object/checkrun/) オブジェクトを参照してください。
-You'll use the [Ruby programming language](https://www.ruby-lang.org/en/), the [Smee](https://smee.io/) webhook payload delivery service, the [Octokit.rb Ruby library](http://octokit.github.io/octokit.rb/) for the GitHub REST API, and the [Sinatra web framework](http://sinatrarb.com/) to create your Checks API CI server app.
+[Ruby プログラミング言語](https://www.ruby-lang.org/en/)、[Smee](https://smee.io/) webhook ペイロード配信サービス、GitHub REST API 用の [Octokit.rb Ruby ライブラリ](http://octokit.github.io/octokit.rb/)、および [Sinatra ウェブフレームワーク](http://sinatrarb.com/) を使用して、Checks API CI サーバーアプリケーションを作成します。
このプロジェクトを完了するために、これらのツールや概念のエキスパートである必要はありません。 このガイドでは、必要なステップを順番に説明していきます。 Checks API で CI テストを作成する前に、以下を行う必要があります。
@@ -70,7 +70,7 @@ You'll use the [Ruby programming language](https://www.ruby-lang.org/en/), the [
### パート1. Checks API インターフェースを作成する
-このパートでは、`check_suite` webhook イベントを受信するために必要なコードを追加し、チェック実行を作成して更新します。 また、GitHub でチェックが再リクエストされた場合にチェック実行を作成する方法についても学びます。 At the end of this section, you'll be able to view the check run you created in a GitHub pull request.
+このパートでは、`check_suite` webhook イベントを受信するために必要なコードを追加し、チェック実行を作成して更新します。 また、GitHub でチェックが再リクエストされた場合にチェック実行を作成する方法についても学びます。 このセクションの最後では、GitHub プルリクエストで作成したチェック実行を表示できるようになります。
このセクションでは、作成したチェック実行はコードでチェックを実行しません。 この機能については、[パート 2: Octo RuboCop CI テストを作成する](#part-2-creating-the-octo-rubocop-ci-test)で追加します。
@@ -383,21 +383,21 @@ RuboCop はコマンドラインユーティリティとして使用できます
require 'git'
```
-リポジトリをクローンするには、アプリケーションに「リポジトリコンテンツ」の読み取り権限が必要です。 このクイックスタートでは、後ほどコンテンツを GitHub にプッシュする必要がありますが、そのためには書き込み権限が必要です。 Go ahead and set your app's "Repository contents" permission to **Read & write** now so you don't need to update it again later. アプリケーションの権限を更新するには、以下の手順に従います。
+リポジトリをクローンするには、アプリケーションに「リポジトリコンテンツ」の読み取り権限が必要です。 このクイックスタートでは、後ほどコンテンツを GitHub にプッシュする必要がありますが、そのためには書き込み権限が必要です。 先に進んでアプリケーションの [Repository contents] の権限を [**Read & write**] に今すぐ変更してください。そうすれば、後で再び変更する必要がなくなります。 アプリケーションの権限を更新するには、以下の手順に従います。
1. [アプリケーションの設定ページ](https://github.com/settings/apps)からアプリケーションを選択肢、サイドバーの [**Permissions & Webhooks**] をクリックします。
-1. In the "Permissions" section, find "Repository contents", and select **Read & write** in the "Access" dropdown next to it.
+1. [Permissions] セクションで [Repository contents] を見つけて、隣にある [Access] ドロップダウンで [**Read & write**] を選択します。
{% data reusables.apps.accept_new_permissions_steps %}
-To clone a repository using your GitHub App's permissions, you can use the app's installation token (`x-access-token:`) shown in the example below:
+GitHub App の権限を用いてリポジトリをクローンするには、以下の例で示すアプリケーションのインストールトークン (`x-access-token:`) を使用できます。
```shell
git clone https://x-access-token:@github.com//.git
```
-The code above clones a repository over HTTP. It requires the full repository name, which includes the repository owner (user or organization) and the repository name. For example, the [octocat Hello-World](https://github.com/octocat/Hello-World) repository has a full name of `octocat/hello-world`.
+上記のコードは、HTTP 経由でリポジトリをクローンします。 コードには、リポジトリの所有者 (ユーザまたは Organization) およびリポジトリ名を含む、リポジトリのフルネームを入力する必要があります。 たとえば、[octocat Hello-World](https://github.com/octocat/Hello-World) リポジトリのフルネームは `octocat/hello-world` です。
-After your app clones the repository, it needs to pull the latest code changes and check out a specific Git ref. The code to do all of this will fit nicely into its own method. To perform these operations, the method needs the name and full name of the repository and the ref to checkout. The ref can be a commit SHA, branch, or tag. Add the following new method to the helper method section in `template_server.rb`:
+アプリケーションがリポジトリをクローンした後は、直近のコード変更をプルし、特定の Git ref をチェックアウトする必要があります。 これら全てを行うコードは、独自のメソッドにするとよいでしょう。 メソッドがこれらの操作を実行するには、リポジトリの名前とフルネーム、チェックアウトする ref が必要です。 ref にはコミット SHA、ブランチ、タグ名を指定できます。 以下の新たなメソッドを `template_server.rb` のヘルパー メソッド セクションに追加します。
``` ruby
# Clones the repository to the current working directory, updates the
@@ -416,11 +416,11 @@ def clone_repository(full_repo_name, repository, ref)
end
```
-The code above uses the `ruby-git` gem to clone the repository using the app's installation token. This code clones the code in the same directory as `template_server.rb`. To run Git commands in the repository, the code needs to change into the repository directory. Before changing directories, the code stores the current working directory in a variable (`pwd`) to remember where to return before exiting the `clone_repository` method.
+上記のコードでは、`ruby-git` gem を使用して、アプリケーションのインストールトークンを使用するリポジトリをクローンします。 このコードは、`template_server.rb` と同じディレクトリ内のコードをクローンします。 リポジトリで Git コマンドを実行するには、コードをリポジトリのディレクトリに変更する必要があります。 ディレクトリを変更する前に、コードはカレントワーキングディレクトリを変数 (`pwd`) に保存して、戻るべき場所を記憶してから、`clone_repository` メソッドを終了します。
-From the repository directory, this code fetches and merges the latest changes (`@git.pull`), checks out the ref (`@git.checkout(ref)`), then changes the directory back to the original working directory (`pwd`).
+このコードは、リポジトリのディレクトリから直近の変更をフェッチしてマージし (`@git.pull`)、ref をチェックアウトし (`@git.checkout(ref)`)、それから元のワーキングディレクトリに戻ります (`pwd`)。
-Now you've got a method that clones a repository and checks out a ref. Next, you need to add code to get the required input parameters and call the new `clone_repository` method. Add the following code under the `***** RUN A CI TEST *****` comment in your `initiate_check_run` helper method:
+さて、これでリポジトリをクローンし、ref をチェックアウトするメソッドができました。 次に、必要な入力パラメータを取得するコードを追加し、新しい `clone_repository` メソッドを呼び出す必要があります。 `initiate_check_run` ヘルパー メソッドの、`***** RUN A CI TEST *****` というコメントの下に、以下のコードを追加します。
``` ruby
# ***** RUN A CI TEST *****
@@ -431,13 +431,13 @@ head_sha = @payload['check_run']['head_sha']
clone_repository(full_repo_name, repository, head_sha)
```
-The code above gets the full repository name and the head SHA of the commit from the `check_run` webhook payload.
+上記のコードは、リポジトリのフルネームとコミットのヘッド SHA を `check_run` webhook ペイロードから取得します。
### ステップ 2.3. RuboCop を実行する
-これでうまくいきました。 You're cloning the repository and creating check runs using your CI server. Now you'll get into the nitty gritty details of the [RuboCop linter](https://rubocop.readthedocs.io/en/latest/basic_usage/#rubocop-as-a-code-style-checker) and [Checks API annotations](/v3/checks/runs/#create-a-check-run).
+これでうまくいきました。 You're cloning the repository and creating check runs using your CI server. それではいよいよ [RuboCop 文法チェッカー](https://rubocop.readthedocs.io/en/latest/basic_usage/#rubocop-as-a-code-style-checker) と [Checks API アノテーション](/v3/checks/runs/#create-a-check-run)の核心に迫ります。
-The following code runs RuboCop and saves the style code errors in JSON format. Add this code below the call to `clone_repository` you added in the [previous step](#step-22-cloning-the-repository) and above the code that updates the check run to complete.
+次のコードは、RuboCop を実行し、スタイル コード エラーを JSON フォーマットで保存します。 Add this code below the call to `clone_repository` you added in the [previous step](#step-22-cloning-the-repository) and above the code that updates the check run to complete.
``` ruby
# Run RuboCop on all files in the repository
@@ -447,23 +447,23 @@ logger.debug @report
@output = JSON.parse @report
```
-The code above runs RuboCop on all files in the repository's directory. The option `--format json` is a handy way to save a copy of the linting results in a machine-parsable format. See the [RuboCop docs](https://rubocop.readthedocs.io/en/latest/formatters/#json-formatter) for details and an example of the JSON format.
+上記のコードは、リポジトリのディレクトリにある全てのファイルで RuboCop を実行します。 `--format json` のオプションは、文法チェックの結果のコピーをコンピューターで読みとることができるフォーマットで保存する便利な方法です。 JSON フォーマットの詳細および例については、[RuboCop ドキュメント](https://rubocop.readthedocs.io/en/latest/formatters/#json-formatter)を参照してください。
-Because this code stores the RuboCop results in a `@report` variable, it can safely remove the checkout of the repository. This code also parses the JSON so you can easily access the keys and values in your GitHub App using the `@output` variable.
+このコードは RuboCop の結果を `@report` 変数に格納するため、リポジトリのチェックアウトを安全に削除できます。 また、このコードは JSON も解析するため、`@output` 変数を使用して GitHub App のキーと変数に簡単にアクセスできます。
{% note %}
-**Note:** The command used to remove the repository (`rm -rf`) cannot be undone. See [Step 2.7. Security tips](#step-27-security-tips) to learn how to check webhooks for injected malicious commands that could be used to remove a different directory than intended by your app. For example, if a bad actor sent a webhook with the repository name `./`, your app would remove the root directory. 😱 If for some reason you're _not_ using the method `verify_webhook_signature` (which is included in `template_server.rb`) to validate the sender of the webhook, make sure you check that the repository name is valid.
+**注釈:** リポジトリを削除するコマンド (`rm -rf`) を使用すると、元に戻すことはできません。 [ステップ 2.7. セキュリティのヒント](#step-27-security-tips)で、webhook を調べて、意図したものとは別のディレクトリを削除するためアプリケーションが使用する可能性のある、インジェクションされた悪意あるコマンドがないかを確認する方法について学びます。 例えば、悪意のある人が `./` というリポジトリ名の webhook を送信した場合、アプリケーションはルートディレクトリを削除するかもしれません。 😱 もし、何らかの理由で webhook の送信者を検証するメソッド `verify_webhook_signature` (`template_server.rb` に含まれています) を使用_しない_場合、リポジトリ名が有効であることを必ず確認するようにしてください。
{% endnote %}
-You can test that this code works and see the errors reported by RuboCop in your server's debug output. Start up the `template_server.rb` server again and create a new pull request in the repository where you're testing your app:
+このコードが動作することをテストし、サーバーのデバッグ出力で RuboCop により報告されたエラーを確認できます。 `template_server.rb` サーバーを再起動し、以下のコマンドで、アプリケーションをテストする場所のリポジトリに新しいプルリクエストを作成します。
```shell
$ ruby template_server.rb
```
-You should see the linting errors in the debug output, although they aren't printed with formatting. You can use a web tool like [JSON formatter](https://jsonformatter.org/) to format your JSON output like this formatted linting error output:
+デバッグ出力に文法エラーが表示されているはずです。ただし、出力は整形されていません。 [JSON フォーマッター](https://jsonformatter.org/)のような Web ツールを使用すると、JSON 出力の文法エラーの出力を以下のように整形できます。
```json
{
@@ -521,15 +521,15 @@ You should see the linting errors in the debug output, although they aren't prin
### ステップ 2.4. RuboCop のエラーを収集する
-The `@output` variable contains the parsed JSON results of the RuboCop report. As shown above, the results contain a `summary` section that your code can use to quickly determine if there are any errors. The following code will set the check run conclusion to `success` when there are no reported errors. RuboCop reports errors for each file in the `files` array, so if there are errors, you'll need to extract some data from the file object.
+`@output` 変数には、RuboCop レポートの解析済み JSON の結果が含まれています。 上記で示す通り、結果には `summary` セクションが含まれており、コードでエラーがあるかどうかを迅速に判断するために使用できます。 以下のコードは、報告されたエラーがない場合に、チェック実行の結果を `success` に設定します。 RuboCop は、`files` 配列内にある各ファイルについてエラーを報告します。エラーがある場合、ファイル オブジェクトからデータを抽出する必要があります。
-The Checks API allows you to create annotations for specific lines of code. When you create or update a check run, you can add annotations. In this quickstart you are [updating the check run](/v3/checks/runs/#update-a-check-run) with annotations.
+Checks API により、コードの特定の行に対してアノテーションを作成することができます。 チェック実行を作成または更新する際に、アノテーションを追加できます。 このクイックスタートでは、アノテーションを付けて[チェック実行を更新](/v3/checks/runs/#update-a-check-run)します。
-The Checks API limits the number of annotations to a maximum of 50 per API request. To create more than 50 annotations, you have to make multiple requests to the [Update a check run](/v3/checks/runs/#update-a-check-run) endpoint. For example, to create 105 annotations you'd need to call the [Update a check run](/v3/checks/runs/#update-a-check-run) endpoint three times. The first two requests would each have 50 annotations, and the third request would include the five remaining annotations. Each time you update the check run, annotations are appended to the list of annotations that already exist for the check run.
+Checks API では、アノテーションの数は API の 1 リクエストあたり最大 50 に制限されています。 51 以上のアノテーションを作成するには、[チェック実行を更新する](/v3/checks/runs/#update-a-check-run)エンドポイントに複数回のリクエストを行う必要があります。 たとえば、105 のアノテーションを作成するには、[チェック実行を更新する](/v3/checks/runs/#update-a-check-run)エンドポイントを 3 回呼び出す必要があります。 始めの 2 回のリクエストでそれぞれ 50 個のアノテーションが作成され、3 回目のリクエストで残り 5 つのアノテーションが作成されます。 チェック実行を更新するたびに、アノテーションは既存のチェック実行にあるアノテーションのリストに追加されます。
-A check run expects annotations as an array of objects. Each annotation object must include the `path`, `start_line`, `end_line`, `annotation_level`, and `message`. RuboCop provides the `start_column` and `end_column` too, so you can include those optional parameters in the annotation. Annotations only support `start_column` and `end_column` on the same line. See the [`annotations` object](/v3/checks/runs/#annotations-object-1) reference documentation for details.
+チェック実行は、アノテーションをオブジェクトの配列として受け取ります。 アノテーションの各オブジェクトには、`path`、`start_line`、 `end_line`、`annotation_level`、`message` を含める必要があります。 RuboCop では `start_column` および `end_column` も提供しており、これらのオプションのパラメータをアノテーションに含めることもできます。 Annotations only support `start_column` and `end_column` on the same line. 詳細については [`annotations` オブジェクト](/v3/checks/runs/#annotations-object-1)のリファレンスドキュメントを参照してください。
-You'll extract the required information from RuboCop needed to create each annotation. Append the following code to the code you added in the [previous section](#step-23-running-rubocop):
+各アノテーションを作成するために必要な RuboCop から、必須の情報を抽出します。 [前のセクション](#step-23-running-rubocop)で追加したコードに、次のコードを追加します。
``` ruby
annotations = []
@@ -584,21 +584,21 @@ else
end
```
-This code limits the total number of annotations to 50. But you can modify this code to update the check run for each batch of 50 annotations. The code above includes the variable `max_annotations` that sets the limit to 50, which is used in the loop that iterates through the offenses.
+このコードでは、アノテーションの合計数を 50 に制限しています。 このコードを、50 のアノテーションごとにチェック実行を更新するよう変更することも可能です。 上記のコードには、制限 を 50 に設定している変数 `max_annotations` が含まれ、これは違反の部分を反復処理するループで使用されています。
-When the `offense_count` is zero, the CI test is a `success`. If there are errors, this code sets the conclusion to `neutral` in order to prevent strictly enforcing errors from code linters. But you can change the conclusion to `failure` if you would like to ensure that the check suite fails when there are linting errors.
+`offense_count` が 0 の場合、CI テストは `success` となります。 エラーがある場合、このコードは結果を `neutral` に設定します。これは、コードの文法チェッカーによるエラーを厳格に強制することを防ぐためです。 ただし、文法エラーがある場合にチェックスイートが失敗になるようにしたい場合は、結果を `failure` に変更できます。
-When errors are reported, the code above iterates through the `files` array in the RuboCop report. For each file, it extracts the file path and sets the annotation level to `notice`. You could go even further and set specific warning levels for each type of [RuboCop Cop](https://rubocop.readthedocs.io/en/latest/cops/), but to keep things simpler in this quickstart, all errors are set to a level of `notice`.
+エラーが報告されると、上記のコードは ReboCop レポートの `files` 配列を反復処理します。 コードは各ファイルにおいてファイルパスを抽出し、アノテーションレベルを `notice` に設定します。 さらに細かく、[RuboCop Cop](https://rubocop.readthedocs.io/en/latest/cops/) の各タイプに特定の警告レベルを設定することもできますが、このクイックスタートでは簡単さを優先し、すべてのエラーを `notice` のレベルに設定します。
-This code also iterates through each error in the `offenses` array and collects the location of the offense and error message. After extracting the information needed, the code creates an annotation for each error and stores it in the `annotations` array. Because annotations only support start and end columns on the same line, `start_column` and `end_column` are only added to the `annotation` object if the start and end line values are the same.
+このコードはまた、`offenses` 配列の各エラーを反復処理し、違反の場所とエラー メッセージを収集します。 必要な情報を抽出後、コードは各エラーに対してアノテーションを作成し、それを `annotations` 配列に格納します。 Because annotations only support start and end columns on the same line, `start_column` and `end_column` are only added to the `annotation` object if the start and end line values are the same.
-This code doesn't yet create an annotation for the check run. You'll add that code in the next section.
+このコードはまだチェック実行のアノテーションを作成しません。 それを作成するコードは、次のセクションで追加します。
### ステップ 2.5. CI テスト結果でチェック実行を更新する
-Each check run from GitHub contains an `output` object that includes a `title`, `summary`, `text`, `annotations`, and `images`. The `summary` and `title` are the only required parameters for the `output`, but those alone don't offer much detail, so this quickstart adds `text` and `annotations` too. The code here doesn't add an image, but feel free to add one if you'd like!
+GitHub から実行される各チェックには、`output` オブジェクトが含まれ、そのオブジェクトには `title`、`summary`、`text`、`annotations`、`images` が含まれます。 `output` に必須のパラメータは `summary` と `title` のみですが、これだけでは詳細情報が得られないので、このクイックスタートでは `text` と `annotations` も追加します。 ここに挙げるコードでは画像は追加しませんが、追加したければぜひどうぞ。
-For the `summary`, this example uses the summary information from RuboCop and adds some newlines (`\n`) to format the output. You can customize what you add to the `text` parameter, but this example sets the `text` parameter to the RuboCop version. To set the `summary` and `text`, append this code to the code you added in the [previous section](#step-24-collecting-rubocop-errors):
+この例では、`summary` は RuboCop からのサマリー情報を利用し、出力を整形するためいくつかの改行 (`\n`) を追加します。 `text` パラメータに何を追加するかはカスタマイズできますが、この例では `text` パラメータを RuboCop のバージョンに設定しています。 `summary` と `text` を設定するには、[前のセクション](#step-24-collecting-rubocop-errors)で追加したコードに、以下のコードを付け加えます。
``` ruby
# Updated check run summary and text parameters
@@ -606,7 +606,7 @@ summary = "Octo RuboCop summary\n-Offense count: #{@output['summary']['offense_c
text = "Octo RuboCop version: #{@output['metadata']['rubocop_version']}"
```
-Now you've got all the information you need to update your check run. In the [first half of this quickstart](#step-14-updating-a-check-run), you added this code to set the status of the check run to `success`:
+さて、これでチェック実行を更新するために必要な情報がすべて揃いました。 [このクイックスタートの前半](#step-14-updating-a-check-run)では、このコードを追加して、チェック実行のステータスを `success` に設定しました。
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
``` ruby
@@ -638,7 +638,7 @@ updated_check_run = @installation_client.patch(
```
{% endif %}
-You'll need to update that code to use the `conclusion` variable you set based on the RuboCop results (to `success` or `neutral`). You can update the code with the following:
+RuboCop の結果に基づいて (`success` または `neutral` に) 設定した `conclusion` 変数を使用するよう、このコードを更新する必要があります。 コードは以下のようにして更新できます。
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
``` ruby
@@ -692,9 +692,9 @@ updated_check_run = @installation_client.patch(
```
{% endif %}
-Now that you're setting a conclusion based on the status of the CI test and you've added the output from the RuboCop results, you've created a CI test! Congratulations. 🙌
+さて、これで CI テストのステータスに基づいて結論を設定し、RuboCop の結果からの出力を追加しました。あなたは CI テストを作成したのです。 おめでとうございます。 🙌
-The code above also adds a feature to your CI server called [requested actions](https://developer.github.com/changes/2018-05-23-request-actions-on-checks/) via the `actions` object. {% if currentVersion == "free-pro-team@latest" %}(Note this is not related to [GitHub Actions](/actions).) {% endif %}Requested actions add a button in the **Checks** tab on GitHub that allows someone to request the check run to take additional action. The additional action is completely configurable by your app. For example, because RuboCop has a feature to automatically fix the errors it finds in Ruby code, your CI server can use a requested actions button to allow people to request automatic error fixes. When someone clicks the button, the app receives the `check_run` event with a `requested_action` action. Each requested action has an `identifier` that the app uses to determine which button was clicked.
+また、上記のコードは、`actions` オブジェクトを介して CI サーバーに[リクエストされたアクション](https://developer.github.com/changes/2018-05-23-request-actions-on-checks/)という機能も追加しています。 {% if currentVersion == "free-pro-team@latest" %}(Note this is not related to [GitHub Actions](/actions).) {% endif %}Requested actions add a button in the **Checks** tab on GitHub that allows someone to request the check run to take additional action. The additional action is completely configurable by your app. For example, because RuboCop has a feature to automatically fix the errors it finds in Ruby code, your CI server can use a requested actions button to allow people to request automatic error fixes. When someone clicks the button, the app receives the `check_run` event with a `requested_action` action. Each requested action has an `identifier` that the app uses to determine which button was clicked.
The code above doesn't have RuboCop automatically fix errors yet. You'll add that in the next section. But first, take a look at the CI test that you just created by starting up the `template_server.rb` server again and creating a new pull request:
@@ -834,7 +834,7 @@ end
Here are a few common problems and some suggested solutions. If you run into any other trouble, you can ask for help or advice in the {% data variables.product.prodname_support_forum_with_url %}.
-* **Q:** My app isn't pushing code to GitHub. I don't see the fixes that RuboCop automatically makes!
+* **Q:** アプリケーションが GitHub にコードをプッシュしません。 I don't see the fixes that RuboCop automatically makes!
**A:** Make sure you have **Read & write** permissions for "Repository contents," and that you are cloning the repository with your installation token. See [Step 2.2. Cloning the repository](#step-22-cloning-the-repository) for details.
diff --git a/translations/ja-JP/content/developers/apps/identifying-and-authorizing-users-for-github-apps.md b/translations/ja-JP/content/developers/apps/identifying-and-authorizing-users-for-github-apps.md
index 8c79d44673d8..94e9798727af 100644
--- a/translations/ja-JP/content/developers/apps/identifying-and-authorizing-users-for-github-apps.md
+++ b/translations/ja-JP/content/developers/apps/identifying-and-authorizing-users-for-github-apps.md
@@ -123,11 +123,13 @@ curl -H "Authorization: token OAUTH-TOKEN" {% data variables.product.api_url_pre
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
### デバイスフロー
+{% if currentVersion ver_lt "enterprise-server@3.1" %}
{% note %}
-**ノート:** デバイスフローはパブリックベータであり、変更されることがあります。{% if currentVersion == "free-pro-team@latest" %} このベータの機能を有効化するには、「[アプリケーションのベータ機能のアクティベート](/developers/apps/activating-beta-features-for-apps)」を参照してください。{% endif %}
+**Note:** The device flow is in public beta and subject to change.
{% endnote %}
+{% endif %}
デバイスフローを使えば、CLIツールやGit認証情報マネージャーなどのヘッドレスアプリケーションのユーザを認可できます。
@@ -904,7 +906,7 @@ While most of your API interaction should occur using your server-to-server inst
* [Get the authenticated user](/v3/users/#get-the-authenticated-user)
* [List app installations accessible to the user access token](/v3/apps/installations/#list-app-installations-accessible-to-the-user-access-token)
{% if currentVersion == "free-pro-team@latest" %}
-* [List subscriptions for the authenticated user](/v3/apps/marketplace/#list-subscriptions-for-the-authenticated-user)
+* [認証されたユーザのサブスクリプションのリスト](/v3/apps/marketplace/#list-subscriptions-for-the-authenticated-user)
{% endif %}
* [List users](/v3/users/#list-users)
* [Get a user](/v3/users/#get-a-user)
diff --git a/translations/ja-JP/content/developers/github-marketplace/setting-pricing-plans-for-your-listing.md b/translations/ja-JP/content/developers/github-marketplace/setting-pricing-plans-for-your-listing.md
index e135da2504bb..98b779092591 100644
--- a/translations/ja-JP/content/developers/github-marketplace/setting-pricing-plans-for-your-listing.md
+++ b/translations/ja-JP/content/developers/github-marketplace/setting-pricing-plans-for-your-listing.md
@@ -65,7 +65,7 @@ versions:
{% data variables.product.prodname_marketplace %}プランの価格プランが不要になった場合、あるいは価格の細部を調整する必要が生じた場合、その価格プランを削除できます。
-
+
{% data variables.product.prodname_marketplace %}にリスト済みのアプリケーションの価格プランを公開すると、そのプランは変更できなくなります。 その代わりに、その価格プランを削除しなければなりません。 削除された価格プランを購入済みの顧客は、オプトアウトして新しい価格プランに移行するまでは、そのプランを使い続けます。 価格プランの詳細については、「[{% data variables.product.prodname_marketplace %}の価格プラン](/marketplace/selling-your-app/github-marketplace-pricing-plans/)」を参照してください。
diff --git a/translations/ja-JP/content/developers/github-marketplace/viewing-metrics-for-your-listing.md b/translations/ja-JP/content/developers/github-marketplace/viewing-metrics-for-your-listing.md
index 597033d417f9..4281e5640dbe 100644
--- a/translations/ja-JP/content/developers/github-marketplace/viewing-metrics-for-your-listing.md
+++ b/translations/ja-JP/content/developers/github-marketplace/viewing-metrics-for-your-listing.md
@@ -1,6 +1,6 @@
---
title: リストのメトリクスの参照
-intro: '{% data variables.product.prodname_marketplace %} インサイトのページは、{% data variables.product.prodname_github_app %}のメトリクスを表示します。 このメトリクスを使って{% data variables.product.prodname_github_app %}のパフォーマンスを追跡し、価格、プラン、無料トライアル、マーケティングキャンペーンの効果の可視化の方法に関する判断を、より多くの情報に基づいて行えます。'
+intro: '{% data variables.product.prodname_marketplace %} Insightsのページは、{% data variables.product.prodname_github_app %}のメトリクスを表示します。 このメトリクスを使って{% data variables.product.prodname_github_app %}のパフォーマンスを追跡し、価格、プラン、無料トライアル、マーケティングキャンペーンの効果の可視化の方法に関する判断を、より多くの情報に基づいて行えます。'
redirect_from:
- /apps/marketplace/managing-github-marketplace-listings/viewing-performance-metrics-for-a-github-marketplace-listing/
- /apps/marketplace/viewing-performance-metrics-for-a-github-marketplace-listing/
@@ -16,38 +16,38 @@ versions:
{% note %}
-**Note:** Because it takes time to aggregate data, you'll notice a slight delay in the dates shown. When you select a time period, you can see exact dates for the metrics at the top of the page.
+**ノート:** データの集計には時間がかかるので、表示される日付には若干の遅れが生じます。 期間を選択すると、ページの上部にそのメトリクスの正確な日付が表示されます。
{% endnote %}
-### Performance metrics
+### パフォーマンスメトリクス
-The Insights page displays these performance metrics, for the selected time period:
+Insightsページには、選択された期間に対する以下のパフォーマンスメトリクスが表示されます。
-* **Subscription value:** Total possible revenue (in US dollars) for subscriptions. This value represents the possible revenue if no plans or free trials are cancelled and all credit transactions are successful. The subscription value includes the full value for plans that begin with a free trial in the selected time period, even when there are no financial transactions in that time period. The subscription value also includes the full value of upgraded plans in the selected time period but does not include the prorated amount. To see and download individual transactions, see "[GitHub Marketplace transactions](/marketplace/github-marketplace-transactions/)."
-* **Visitors:** Number of people that have viewed a page in your GitHub Apps listing. This number includes both logged in and logged out visitors.
-* **Pageviews:** Number of views the pages in your GitHub App's listing received. A single visitor can generate more than one pageview.
+* **Subscription value:** サブスクリプションで可能な合計収入(米ドル)。 この値は、プランや無料トライアルがまったくキャンセルされず、すべてのクレジット取引が成功した場合に可能な収入を示します。 subscription valueには、選択された期間内に無料トライアルで始まったプランの全額が、仮にその期間に金銭取引がなかったとしても含まれます。 subscription valueには、選択された期間内にアップグレードされたプランの全額も含まれますが、日割り計算の文は含まれません。 個別の取引を見てダウンロードするには、「[GitHub Marketplaceの取引](/marketplace/github-marketplace-transactions/)」を参照してください。
+* **Visitors:** GitHub Appのリスト内のページを見た人数。 この数字には、ログインした訪問者とログアウトした訪問者がどちらも含まれます。
+* **Pageviews:** GitHub Appのリスト内のページが受けた閲覧数です。 一人の訪問者が複数のページビューを生成できます。
{% note %}
-**Note:** Your estimated subscription value could be much higher than the transactions processed for this period.
+**ノート:** 推定されたsubscription valueは、その期間に処理された取引よりもはるかに高くなることがあります。
{% endnote %}
-#### Conversion performance
+#### コンバージョンパフォーマンス
-* **Unique visitors to landing page:** Number of people who viewed your GitHub App's landing page.
-* **Unique visitors to checkout page:** Number of people who viewed one of your GitHub App's checkout pages.
-* **Checkout page to new subscriptions:** Total number of paid subscriptions, free trials, and free subscriptions. See the "Breakdown of total subscriptions" for the specific number of each type of subscription.
+* **Unique visitors to landing page:** GitHub Appのランディングページを閲覧した人数。
+* **Unique visitors to checkout page:** GitHub Appのチェックアウトページのいずれかを閲覧した人数。
+* **Checkout page to new subscriptions:** 有料サブスクリプション、無料トライアル、無料サブスクリプションの総数。 それぞれの種類のサブスクリプションの特定の数値については「合計サブスクリプションの内訳」を参照してください。

-To access {% data variables.product.prodname_marketplace %} Insights:
+{% data variables.product.prodname_marketplace %} Insightsには以下のようにしてアクセスしてください。
{% data reusables.user-settings.access_settings %}
{% data reusables.user-settings.developer_settings %}
{% data reusables.user-settings.marketplace_apps %}
-4. {% data variables.product.prodname_github_app %} that you'd like to view Insights for.
+4. Insightを表示させたい{% data variables.product.prodname_github_app %}を選択してください。
{% data reusables.user-settings.edit_marketplace_listing %}
-6. Click the **Insights** tab.
-7. Optionally, select a different time period by clicking the Period dropdown in the upper-right corner of the Insights page. 
+6. **Insights**タブをクリックしてください。
+7. Insightsページの右上にあるPeriod(期間)ドロップダウンをクリックして、異なる期間を選択することもできます。 
diff --git a/translations/ja-JP/content/developers/github-marketplace/viewing-transactions-for-your-listing.md b/translations/ja-JP/content/developers/github-marketplace/viewing-transactions-for-your-listing.md
index 4d9660677e28..a86552796533 100644
--- a/translations/ja-JP/content/developers/github-marketplace/viewing-transactions-for-your-listing.md
+++ b/translations/ja-JP/content/developers/github-marketplace/viewing-transactions-for-your-listing.md
@@ -1,6 +1,6 @@
---
-title: Viewing transactions for your listing
-intro: 'The {% data variables.product.prodname_marketplace %} transactions page allows you to download and view all transactions for your {% data variables.product.prodname_marketplace %} listing. You can view transations for the past day (24 hours), week, month, or for the entire duration of time that your {% data variables.product.prodname_github_app %} has been listed.'
+title: リストの取引の表示
+intro: '{% data variables.product.prodname_marketplace %}の取引ページでは、{% data variables.product.prodname_marketplace %}リストのすべての取引をダウンロードしたり表示したりできます。 過去の日(24時間)、週、月、あるいは{% data variables.product.prodname_github_app %}がリストされた期間全体に対する取引を見ることができます。'
redirect_from:
- /marketplace/github-marketplace-transactions
versions:
@@ -11,35 +11,35 @@ versions:
{% note %}
-**Note:** Because it takes time to aggregate data, you'll notice a slight delay in the dates shown. When you select a time period, you can see exact dates for the metrics at the top of the page.
+**ノート:** データの集計には時間がかかるので、表示される日付には若干の遅れが生じます。 期間を選択すると、ページの上部にそのメトリクスの正確な日付が表示されます。
{% endnote %}
-You can view or download the transaction data to keep track of your subscription activity. Click the **Export CSV** button to download a `.csv` file. You can also select a period of time to view and search within the transaction page.
+サブスクリプションのアクティビティを追跡するために、取引のデータを表示したりダウンロードしたりできます。 **Export CSV(CSVのエクスポート)**ボタンをクリックして、`.csv`ファイルをダウンロードしてください。 取引ページ内で表示したり検索したりする期間を選択することもできます。
-### Transaction data fields
+### 取引のデータフィールド
-* **date:** The date of the transaction in `yyyy-mm-dd` format.
-* **app_name:** The app name.
-* **user_login:** The login of the user with the subscription.
-* **user_id:** The id of the user with the subscription.
-* **user_type:** The type of GitHub account, either `User` or `Organization`.
-* **country:** The three letter country code.
-* **amount_in_cents:** The amount of the transaction in cents. When a value is less the plan amount, the user upgraded and the new plan is prorated. A value of zero indicates the user cancelled their plan.
-* **renewal_frequency:** The subscription renewal frequency, either `Monthly` or `Yearly`.
-* **marketplace_listing_plan_id:** The `id` of the subscription plan.
+* **date:** `yyyy-mm-dd`という形式の取引の日付。
+* **app_name:** アプリケーションの名前。
+* **user_login:** サブスクリプションを持つユーザのログイン。
+* **user_id:** サブスクリプションを持つユーザのID。
+* **user_type:** GitHubアカウントの種類。`User`もしくは`Organization`。
+* **country:** 3文字の国コード。
+* **amount_in_cents:** セント単位での取引の額。 値がプランの額を下回っている場合は、ユーザがアップグレードをして新しいプランが日割りになっています。 値がゼロになっている場合は、ユーザがプランをキャンセルしたことを示します。
+* **renewal_frequency:** サブスクリプションの更新の頻度で、`Monthly`もしくは`Yearly`です。
+* **marketplace_listing_plan_id:** サブスクリプションプランの`id`です。

-### Accessing {% data variables.product.prodname_marketplace %} transactions
+### {% data variables.product.prodname_marketplace %}の取引へのアクセス
-To access {% data variables.product.prodname_marketplace %} transactions:
+{% data variables.product.prodname_marketplace %}の取引にアクセスするには以下のようにしてください。
{% data reusables.user-settings.access_settings %}
{% data reusables.user-settings.developer_settings %}
{% data reusables.user-settings.marketplace_apps %}
-4. {% data variables.product.prodname_github_app %} that you'd like to view transactions for.
+4. 取引を表示させたい{% data variables.product.prodname_github_app %}を選択してください。
{% data reusables.user-settings.edit_marketplace_listing %}
-6. Click the **Transactions** tab.
-7. Optionally, select a different time period by clicking the Period dropdown in the upper-right corner of the Transactions page. 
+6. **Transactions(取引)**タブをクリックしてください。
+7. 取引ページの右上にあるPeriod(期間)ドロップダウンをクリックして、異なる期間を選択することもできます。 
diff --git a/translations/ja-JP/content/developers/github-marketplace/webhook-events-for-the-github-marketplace-api.md b/translations/ja-JP/content/developers/github-marketplace/webhook-events-for-the-github-marketplace-api.md
index 4c45d9bd0794..775b4a70e5d3 100644
--- a/translations/ja-JP/content/developers/github-marketplace/webhook-events-for-the-github-marketplace-api.md
+++ b/translations/ja-JP/content/developers/github-marketplace/webhook-events-for-the-github-marketplace-api.md
@@ -1,6 +1,6 @@
---
-title: Webhook events for the GitHub Marketplace API
-intro: 'A {% data variables.product.prodname_marketplace %} app receives information about changes to a user''s plan from the Marketplace purchase event webhook. A Marketplace purchase event is triggered when a user purchases, cancels, or changes their payment plan. For details on how to respond to each of these types of events, see "[Billing flows](/marketplace/integrating-with-the-github-marketplace-api/#billing-flows)."'
+title: GitHub Marketplace APIのためのwebhookイベント
+intro: '{% data variables.product.prodname_marketplace %}アプリケーションは、ユーザのプランに対する変更に関する情報を、Marketplaceの購入イベントwebhookから受け取ります。 Marketplaceの購入イベントは、ユーザが支払いプランの購入、キャンセル、変更をした場合にトリガーされます。 それぞれの種類のイベントへの対応方法の詳細については「[支払いフロー](/marketplace/integrating-with-the-github-marketplace-api/#billing-flows)」を参照してください。'
redirect_from:
- /apps/marketplace/setting-up-github-marketplace-webhooks/about-webhook-payloads-for-a-github-marketplace-listing/
- /apps/marketplace/integrating-with-the-github-marketplace-api/github-marketplace-webhook-events/
@@ -11,63 +11,63 @@ versions:
-### {% data variables.product.prodname_marketplace %} purchase webhook payload
+### {% data variables.product.prodname_marketplace %}購入webhookのペイロード
-Webhooks `POST` requests have special headers. See "[Webhook delivery headers](/webhooks/event-payloads/#delivery-headers)" for more details. GitHubは、失敗した配信の試行を再送信しません。 GitHubが送信したすべてのwebhookのペイロードを、アプリケーションが確実に受信できるようにしてください。
+webhookの`POST`リクエストには、特別なヘッダがあります。 詳細については「[webhookの配信ヘッダ](/webhooks/event-payloads/#delivery-headers)」を参照してください。 GitHubは、失敗した配信の試行を再送信しません。 GitHubが送信したすべてのwebhookのペイロードを、アプリケーションが確実に受信できるようにしてください。
-Cancellations and downgrades take effect on the first day of the next billing cycle. Events for downgrades and cancellations are sent when the new plan takes effect at the beginning of the next billing cycle. Events for new purchases and upgrades begin immediately. Use the `effective_date` in the webhook payload to determine when a change will begin.
+キャンセル及びダウングレードは、次の支払いサイクルの初日に有効になります。 ダウングレードとキャンセルのイベントは、次の支払いサイクルの開始時に新しいプランが有効になったときに送信されます。 新規の購入とアップグレードのイベントは、すぐに開始されます。 変更がいつ始まるかを判断するには、webhookのペイロード中の`effective_date`を使ってください。
{% data reusables.marketplace.marketplace-malicious-behavior %}
-Each `marketplace_purchase` webhook payload will have the following information:
-
-
-| キー | 種類 | 説明 |
-| ---------------------- | -------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
-| `action` | `string` | The action performed to generate the webhook. Can be `purchased`, `cancelled`, `pending_change`, `pending_change_cancelled`, or `changed`. For more information, see the example webhook payloads below. **Note:** The `pending_change` and `pending_change_cancelled` payloads contain the same keys as shown in the [`changed` payload example](#example-webhook-payload-for-a-changed-event). |
-| `effective_date` | `string` | The date the `action` becomes effective. |
-| `sender` | `オブジェクト` | The person who took the `action` that triggered the webhook. |
-| `marketplace_purchase` | `オブジェクト` | The {% data variables.product.prodname_marketplace %} purchase information. |
-
-The `marketplace_purchase` object has the following keys:
-
-| キー | 種類 | 説明 |
-| -------------------- | --------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `アカウント` | `オブジェクト` | The `organization` or `user` account associated with the subscription. Organization accounts will include `organization_billing_email`, which is the organization's administrative email address. To find email addresses for personal accounts, you can use the [Get the authenticated user](/v3/users/#get-the-authenticated-user) endpoint. |
-| `billing_cycle` | `string` | Can be `yearly` or `monthly`. When the `account` owner has a free GitHub plan and has purchased a free {% data variables.product.prodname_marketplace %} plan, `billing_cycle` will be `nil`. |
-| `unit_count` | `integer` | Number of units purchased. |
-| `on_free_trial` | `boolean` | `true` when the `account` is on a free trial. |
-| `free_trial_ends_on` | `string` | The date the free trial will expire. |
-| `next_billing_date` | `string` | The date that the next billing cycle will start. When the `account` owner has a free GitHub.com plan and has purchased a free {% data variables.product.prodname_marketplace %} plan, `next_billing_date` will be `nil`. |
-| `plan` | `オブジェクト` | The plan purchased by the `user` or `organization`. |
-
-The `plan` object has the following keys:
-
-| キー | 種類 | 説明 |
-| ------------------------ | ------------------ | ------------------------------------------------------------------------------------------------------------------------------------- |
-| `id` | `integer` | The unique identifier for this plan. |
-| `name` | `string` | The plan's name. |
-| `説明` | `string` | This plan's description. |
-| `monthly_price_in_cents` | `integer` | The monthly price of this plan in cents (US currency). For example, a listing that costs 10 US dollars per month will be 1000 cents. |
-| `yearly_price_in_cents` | `integer` | The yearly price of this plan in cents (US currency). For example, a listing that costs 100 US dollars per month will be 10000 cents. |
-| `price_model` | `string` | The pricing model for this listing. Can be one of `flat-rate`, `per-unit`, or `free`. |
-| `has_free_trial` | `boolean` | `true` when this listing offers a free trial. |
-| `unit_name` | `string` | The name of the unit. If the pricing model is not `per-unit` this will be `nil`. |
-| `bullet` | `array of strings` | The names of the bullets set in the pricing plan. |
+それぞれの`marketplace_purchase` webhookのペイロードは、以下の情報を持ちます。
+
+
+| キー | 種類 | 説明 |
+| ---------------------- | -------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| `action` | `string` | webhookを生成するために行われたアクション。 `purchased`、`cancelled`、`pending_change`、`pending_change_cancelled`、`changed`のいずれかになります。 詳しい情報については、以下のwebhookペイロードの例を参照してください。 **ノート:** `pending_change`及び`pending_change_cancelled`ペイロードには、[`changed`ペイロードの例](#example-webhook-payload-for-a-changed-event)に示されているものと同じキーが含まれます。 |
+| `effective_date` | `string` | `action`が有効になる日付。 |
+| `sender` | `object` | webhookをトリガーした`action`を行った人。 |
+| `marketplace_purchase` | `object` | {% data variables.product.prodname_marketplace %}の購入情報。 |
+
+`marketplace_purchase`オブジェクトは、以下のキーを持ちます。
+
+| キー | 種類 | 説明 |
+| -------------------- | --------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `account` | `object` | サブスクリプションに関連づけられた`organization`もしくあは`user`アカウント。 Organizationアカウントは、そのOrganizationの管理者のメールアドレスである`organization_billing_email`を含みます。 個人アカウントのメールアドレスを知るには、[認証されたユーザの取得](/v3/users/#get-the-authenticated-user)エンドポイントが利用できます。 |
+| `billing_cycle` | `string` | `yearly`もしくは`monthly`のいずれかになります。 `account`の所有者が無料のGitHubのプランを使っており、無料の{% data variables.product.prodname_marketplace %}プランを購入した場合、`billing_cycle`は`nil`になります。 |
+| `unit_count` | `integer` | 購入したユーザ数。 |
+| `on_free_trial` | `boolean` | `account`が無料トライアル中の場合`true`になります。 |
+| `free_trial_ends_on` | `string` | 無料トライアルが期限切れになる日付。 |
+| `next_billing_date` | `string` | 次の支払いサイクルが始まる日付。 `account`の所有者が無料のGitHub.comのプランを使っており、無料の{% data variables.product.prodname_marketplace %}プランを購入した場合、`next_billing_date`は`nil`になります。 |
+| `plan` | `オブジェクト` | `user`または`organization`が購入したプラン。 |
+
+`plan`オブジェクトには以下のキーがあります。
+
+| キー | 種類 | 説明 |
+| ------------------------ | ------------------ | ------------------------------------------------------ |
+| `id` | `integer` | このプランの一意の識別子。 |
+| `name` | `string` | プラン名。 |
+| `説明` | `string` | プランの説明。 |
+| `monthly_price_in_cents` | `integer` | このプランのセント (米国の通貨) 単位の月額。 たとえば、月額10米ドルのリストは1000セントです。 |
+| `yearly_price_in_cents` | `integer` | このプランのセント (米国の通貨) 単位の年額。 たとえば、月額100米ドルのリストは10000セントです。 |
+| `price_model` | `string` | このリストの価格モデル。 `flat-rate`、`per-unit`、`free`のいずれかです。 |
+| `has_free_trial` | `boolean` | このリストが無料トライアルを提供する場合は`true`になります。 |
+| `unit_name` | `string` | ユニットの名前。 価格モデルが`per-unit`でない場合、これは`nil`になります。 |
+| `bullet` | `array of strings` | 価格プランに設定されている箇条書きの名前。 |
-#### Example webhook payload for a `purchased` event
-This example provides the `purchased` event payload.
+#### `purchased`イベントのサンプルwebhookペイロード
+次の例は、`purchased`イベントのペイロードを示しています。
{{ webhookPayloadsForCurrentVersion.marketplace_purchase.purchased }}
-#### Example webhook payload for a `changed` event
+#### `changed`イベントのサンプルwebhookペイロード
-Changes in a plan include upgrades and downgrades. This example represents the `changed`,`pending_change`, and `pending_change_cancelled` event payloads. The action identifies which of these three events has occurred.
+プランの変更には、アップグレードとダウンロードがあります。 この例は、`changed`、`pending_change`、および`pending_change_cancelled`イベントのペイロードを表しています。 このアクションは、これら3つのイベントのうちどれが発生したかを示します。
{{ webhookPayloadsForCurrentVersion.marketplace_purchase.changed }}
-#### Example webhook payload for a `cancelled` event
+#### `cancelled`イベントのサンプルwebhookペイロード
{{ webhookPayloadsForCurrentVersion.marketplace_purchase.cancelled }}
diff --git a/translations/ja-JP/content/developers/github-marketplace/writing-a-listing-description-for-your-app.md b/translations/ja-JP/content/developers/github-marketplace/writing-a-listing-description-for-your-app.md
index b11b8f9d2b46..0ea30a12c5e8 100644
--- a/translations/ja-JP/content/developers/github-marketplace/writing-a-listing-description-for-your-app.md
+++ b/translations/ja-JP/content/developers/github-marketplace/writing-a-listing-description-for-your-app.md
@@ -1,6 +1,6 @@
---
-title: Writing a listing description for your app
-intro: 'To [list your app](/marketplace/listing-on-github-marketplace/) in the {% data variables.product.prodname_marketplace %}, you''ll need to write descriptions of your app and provide images that follow GitHub''s guidelines.'
+title: アプリケーションのリストの説明を書く
+intro: '{% data variables.product.prodname_marketplace %}で[アプリケーションをリスト](/marketplace/listing-on-github-marketplace/) するには、GitHubのガイドラインに従ってアプリケーションの説明を書き、画像を指定する必要があります。'
redirect_from:
- /apps/marketplace/getting-started-with-github-marketplace-listings/guidelines-for-writing-github-app-descriptions/
- /apps/marketplace/creating-and-submitting-your-app-for-approval/writing-github-app-descriptions/
@@ -16,180 +16,180 @@ versions:
-Here are guidelines about the fields you'll need to fill out in the **Listing description** section of your draft listing.
+ドラフトリストの [**リストの説明**] セクションに入力する必要があるフィールドについてのガイドラインは以下のとおりです。
-### Naming and links
+### 名前のリンク
-#### Listing name
+#### リスト名
-Your app's name will appear on the [{% data variables.product.prodname_marketplace %} homepage](https://github.com/marketplace). The name is limited to 255 characters.
+アプリケーションの名前は、[{% data variables.product.prodname_marketplace %} ホームページ](https://github.com/marketplace)に表示されます。 名前の上限は 255 文字です。
-#### Very short description
+#### ごく簡単な説明
-The community will see the "very short" description under your app's name on the [{% data variables.product.prodname_marketplace %} homepage](https://github.com/marketplace).
+コミュニティには、[{% data variables.product.prodname_marketplace %}ホームページ](https://github.com/marketplace)のアプリケーション名の下に「ごく短い」説明が表示されます。
-
+
-##### Length
+##### 長さ
-We recommend keeping short descriptions to 40-80 characters. Although you are allowed to use more characters, concise descriptions are easier for customers to read and understand quickly.
+簡単な説明は、40~80文字にとどめることをお勧めします。 それ以上の文字数を使うこともできますが、説明は簡潔なほうが顧客に読みやすく、わかりやすくなります。
-##### Content
+##### 内容
-- Describe the app’s functionality. Don't use this space for a call to action. 例:
+- アプリケーションの機能を説明します。 このスペースを操作の指示には使用しないでください。 例:
- **DO:** Lightweight project management for GitHub issues
+ **良い例:** GitHub Issueの軽量なプロジェクト管理
- **DON'T:** Manage your projects and issues on GitHub
+ **悪い例:** GitHubでプロジェクトとIssueを管理してください
- **Tip:** Add an "s" to the end of the verb in a call to action to turn it into an acceptable description: _Manages your projects and issues on GitHub_
+ **ヒント:** 「~してください」ではなく「~します」と書けば、一応、説明として許容されます。例: _GitHubでプロジェクトとIssueを管理します_
-- Don’t repeat the app’s name in the description.
+- 説明でアプリケーション名は繰り返さないようにします。
- **DO:** A container-native continuous integration tool
+ **良い例:** コンテナ対応の継続的インテグレーションツール
- **DON'T:** Skycap is a container-native continuous integration tool
+ **悪い例:** Skycapは、コンテナ対応の継続的インテグレーションツールです
-##### Formatting
+##### フォーマット
-- Always use sentence-case capitalization. Only capitalize the first letter and proper nouns.
+- 英文字表記は固有名詞に使用し、大文字小文字は常に正しく使ってください。 大文字で始めて英文字表記するのは固有名詞だけです。
-- Don't use punctuation at the end of your short description. Short descriptions should not include complete sentences, and definitely should not include more than one sentence.
+- 短い説明の終わりには句読点を付けません。 完全文では書かないようにし、複数文は絶対に避けてください。
-- Only capitalize proper nouns. 例:
+- 大文字で始めて英文字表記するのは固有名詞だけです。 例:
- **DO:** One-click delivery automation for web developers
+ **良い例:** Web開発者向けのワンクリック配信の自動化
- **DON'T:** One-click delivery automation for Web Developers
+ **悪い例:** Web Developer用のワンクリック配信の自動化
-- Always use a [serial comma](https://en.wikipedia.org/wiki/Serial_comma) in lists.
+- 3つ以上の項目を並べるとき、[最後の「および」の前には読点](https://en.wikipedia.org/wiki/Serial_comma)を打ちます。
-- Avoid referring to the GitHub community as "users."
+- GitHubコミュニティを「ユーザー」と称するのは避けてください。
- **DO:** Create issues automatically for people in your organization
+ **良い例:** Organizationの人に自動的にIssueを作成する
- **DON'T:** Create issues automatically for an organization's users
+ **悪い例:** 組織のユーザーについて自動的にIssueを作成する
-- Avoid acronyms unless they’re well established (such as API). 例:
+- 略語は一般的な場合 (APIなど) を除いて使用しないでください。 例:
- **DO:** Agile task boards, estimates, and reports without leaving GitHub
+ **良い例:** GitHubから移動しないアジャイルタスクボード、推定、およびレポート
- **DON'T:** Agile task boards, estimates, and reports without leaving GitHub’s UI
+ **悪い例'T:** GitHub UIから移動しないアジャイルタスクボード、推定、およびレポート
#### カテゴリ
-Apps in {% data variables.product.prodname_marketplace %} can be displayed by category. Select the category that best describes the main functionality of your app in the **Primary category** dropdown, and optionally select a **Secondary category** that fits your app.
+{% data variables.product.prodname_marketplace %}のアプリケーションはカテゴリ別に表示できます。 アプリケーションの主な機能を端的に表すカテゴリを [**Primary category**] ドロップダウンで選択し、オプションでstrong x-id="1">アプリケーションに適した [**Secondary category**] を選択します。
#### サポートされている言語
-If your app only works with specific languages, select up to 10 programming languages that your app supports. These languages are displayed on your app's {% data variables.product.prodname_marketplace %} listing page. This field is optional.
+アプリケーションが特定の言語でのみ動作する場合は、アプリケーションがサポートしている言語を最大10まで選択します。 選択した言語はアプリケーションの{% data variables.product.prodname_marketplace %}リストページに表示されます。 このフィールドはオプションです。
-#### Listing URLs
+#### URLのリスト
-**Required URLs**
-* **Customer support URL:** The URL of a web page that your customers will go to when they have technical support, product, or account inquiries.
-* **Privacy policy URL:** The web page that displays your app's privacy policy.
-* **Installation URL:** This field is shown for OAuth Apps only. (GitHub Apps don't use this URL because they use the optional Setup URL from the GitHub App's settings page instead.) When a customer purchases your OAuth App, GitHub will redirect customers to the installation URL after they install the app. You will need to redirect customers to `https://github.com/login/oauth/authorize` to begin the OAuth authorization flow. See "[New purchases for OAuth Apps](/marketplace/integrating-with-the-github-marketplace-api/handling-new-purchases-and-free-trials/)" for more details. Skip this field if you're listing a GitHub App.
+**必須のURL**
+* **カスタマーサポートのURL:** 顧客がテクニカルサポート、製品、またはアカウントについて問い合わせるためにアクセスするWebページのURL。
+* **プライバシーポリシーのURL:** アプリケーションのプライバシーポリシーが表示されるWebページ。
+* **インストールURL:** このフィールドが表示されるのはOAuthアプリケーションの場合のみです。 (GitHub AppはこのURLを使用しません。かわりに、GitHub Appの設定ページからオプションの設定URLを使用するからです。) 顧客がOAuth Appを購入する際、アプリケーションのインストール後にGitHubは顧客をインストールURLにリダイレクトします。 OAuth認証フローを始めるには、顧客を`https://github.com/login/oauth/authorize`にリダイレクトする必要があります。 詳細については、「[OAuthアプリケーションの新規購入](/marketplace/integrating-with-the-github-marketplace-api/handling-new-purchases-and-free-trials/)」を参照してください。 GitHub Appをリストする場合、このフィールドはスキップしてください。
-**Optional URLs**
-* **Company URL:** A link to your company's website.
-* **Status URL:** A link to a web page that displays the status of your app. Status pages can include current and historical incident reports, web application uptime status, and scheduled maintenance.
-* **Documentation URL:** A link to documentation that teaches customers how to use your app.
+**オプションのURL**
+* **企業URL:** 会社のWebサイトへのリンク。
+* **ステータスURL:** アプリケーションのステータスを表示するWebページへのリンク。 ステータスページには、現在および履歴のインシデントレポート、Webアプリケーションの稼働時間ステータス、およびメンテナンスのスケジュールが記載されます。
+* **ドキュメントのURL:** 顧客にアプリケーションの使用方法を説明するドキュメントへのリンク。
-### Logo and feature card
+### ロゴと機能カード
-{% data variables.product.prodname_marketplace %} displays all listings with a square logo image inside a circular badge to visually distinguish apps.
+{% data variables.product.prodname_marketplace %}には、アプリケーションを視覚的に区別するために、円形のバッジの中に四角いロゴ画像の付いたリストが表示されます。
-
+
-A feature card consists of your app's logo, name, and a custom background image that captures your brand personality. {% data variables.product.prodname_marketplace %} displays this card if your app is one of the four randomly featured apps at the top of the [homepage](https://github.com/marketplace). Each app's very short description is displayed below its feature card.
+機能カードは、アプリケーションのロゴ、名前、およびブランドの個性を捉えた顧客の背景画像で構成されます。 アプリケーションが、ランダムに選択されて[ホームページ](https://github.com/marketplace)の上部に表示されているアプリケーションの1つである場合、{% data variables.product.prodname_marketplace %}には、このカードが表示されます。 各アプリケーションのごく短い説明が、機能カードの下に表示されます。
-
+
-As you upload images and select colors, your {% data variables.product.prodname_marketplace %} draft listing will display a preview of your logo and feature card.
+画像をアップロードして色を選択すると、{% data variables.product.prodname_marketplace %}のドラフトリストに、ロゴと機能カードのプレビューが表示されます。
-##### Guidelines for logos
+##### ロゴのガイドライン
-You must upload a custom image for the logo. For the badge, choose a background color.
+ロゴ用として、カスタム画像をアップロードする必要があります。 バッジには、背景色を選択します。
-- Upload a logo image that is at least 200 pixels x 200 pixels so your logo won't have to be upscaled when your listing is published.
-- Logos will be cropped to a square. We recommend uploading a square image file with your logo in the center.
-- For best results, upload a logo image with a transparent background.
-- To give the appearance of a seamless badge, choose a badge background color that matches the background color (or transparency) of your logo image.
-- Avoid using logo images with words or text in them. Logos with text do not scale well on small screens.
+- リストを公開するときにアップスケールしなくて済むように、アップロードするロゴ画像は200×200ピクセル以上にしてください。
+- ロゴは正方形にトリミングされます。 ロゴが中央にある正方形の画像ファイルをアップロードすることをお勧めします。
+- 最適な結果を得るには、透明な背景のロゴ画像をアップロードしてください。
+- 継ぎ目がないようにバッジを表示するには、ロゴ画像の背景色 (または透明) と一致する色をバッジの背景として選択します。
+- 単語や文章が含まれるロゴ画像の使用は避けてください。 文字が含まれる画像は、小さい画面で適切に縮小されません。
-##### Guidelines for feature cards
+##### 機能カードのガイドライン
-You must upload a custom background image for the feature card. For the app's name, choose a text color.
+機能カード用として、カスタム背景画像をアップロードする必要があります。 アプリケーションの名前には、文字色を選択します。
-- Use a pattern or texture in your background image to give your card a visual identity and help it stand out against the dark background of the {% data variables.product.prodname_marketplace %} homepage. Feature cards should capture your app's brand personality.
-- Background image measures 965 pixels x 482 pixels (width x height).
-- Choose a text color for your app's name that shows up clearly over the background image.
+- カードを視覚的に区別しやすいように、また{% data variables.product.prodname_marketplace %}ホームページの暗い背景に対して目立つように、背景画像にはパターンまたはテクスチャを使用します。 機能カードには、アプリケーションのブランドの個性を取得する必要があります。
+- 背景画像の大きさは、幅965ピクセル x 高さ482ピクセルです。
+- アプリケーション名の文字色には、背景画像に対してはっきり見える色を選択してください。
-### Listing details
+### リストの詳細
-To get to your app's landing page, click your app's name from the {% data variables.product.prodname_marketplace %} homepage or category page. The landing page displays a longer description of the app, which includes two parts: an "Introductory description" and a "Detailed description."
+アプリケーションのランディングページにアクセスするには、{% data variables.product.prodname_marketplace %}ホームページまたはカテゴリページからアプリケーションの名前をクリックします。 ランディングページページには、アプリケーションの長い説明が表示されます。説明は「概要説明」と「詳細説明」の2部で構成されています。
-Your "Introductory description" is displayed at the top of your app's {% data variables.product.prodname_marketplace %} landing page.
+「概要説明」は、アプリケーションの{% data variables.product.prodname_marketplace %}ランディングページの上部に表示されます。
-
+
-Clicking **Read more...**, displays the "Detailed description."
+[**Read more...**] をクリックすると、「詳細説明」が表示されます。
-
+
-Follow these guidelines for writing these descriptions.
+説明の記述は、以下のガイドラインに従ってください。
-#### Length
+#### 長さ
-We recommend writing a 1-2 sentence high-level summary between 150-250 characters in the required "Introductory description" field when [listing your app](/marketplace/listing-on-github-marketplace/). Although you are allowed to use more characters, concise summaries are easier for customers to read and understand quickly.
+[アプリケーションをリストする](/marketplace/listing-on-github-marketplace/)ときの必須の「概要説明」フィールドに、150~250文字の長さで1、2文くらいの概要を記述してください。 それ以上の文字数を使うこともできますが、概要は簡潔なほうが顧客に読みやすく、わかりやすくなります。
-You can add more information in the optional "Detailed description" field. You see this description when you click **Read more...** below the introductory description on your app's landing page. A detailed description consists of 3-5 [value propositions](https://en.wikipedia.org/wiki/Value_proposition), with 1-2 sentences describing each one. You can use up to 1,000 characters for this description.
+オプションの「詳細説明」フィールドに情報を追加することもできます。 アプリケーションのランディングページで概要説明の下にある [**Read more...**] をクリックすると、この説明が表示されます。 詳細説明は3~5個の[バリュープロポジション](https://en.wikipedia.org/wiki/Value_proposition)で構成され、それぞれが1、2文の説明です。 この説明には、最大1,000文字まで使用できます。
-#### Content
+#### 内容
-- Always begin introductory descriptions with your app's name.
+- 概要説明は、必ずアプリケーション名から始めます。
-- Always write descriptions and value propositions using the active voice.
+- 説明とバリュープロポジションは、必ず能動態で書きます。
-#### Formatting
+#### フォーマット
-- Always use sentence-case capitalization in value proposition titles. Only capitalize the first letter and proper nouns.
+- バリュープロポジションでは、英文字表記は固有名詞に使用し、大文字小文字は常に正しく使ってください。 大文字で始めて英文字表記するのは固有名詞だけです。
-- Use periods in your descriptions. Avoid exclamation marks.
+- 説明では句点を使用します。 感嘆符は避けてください。
-- Don't use punctuation at the end of your value proposition titles. Value proposition titles should not include complete sentences, and should not include more than one sentence.
+- バリュープロポジションのタイトルの終わりには句読点を付けません。 バリュープロポジションのタイトルを完全文では書かないようにし、複数文は避けてください。
-- For each value proposition, include a title followed by a paragraph of description. Format the title as a [level-three header](/articles/basic-writing-and-formatting-syntax/#headings) using Markdown. 例:
+- 各バリュープロポジションには、タイトルとそれに続く説明があります。 タイトルは、Markdownを使用して[レベル3ヘッダ](/articles/basic-writing-and-formatting-syntax/#headings)としてフォーマットします。 例:
- ### Learn the skills you need
+ ### 必要なスキルを学ぶ
- GitHub Learning Lab can help you learn how to use GitHub, communicate more effectively with Markdown, handle merge conflicts, and more.
+ GitHub Learning Labでは、GitHubの使い方を学習、Markdownによる効果的な連絡、マージコンフリクトの処理などが可能です。
-- Only capitalize proper nouns.
+- 大文字で始めて英文字表記するのは固有名詞だけです。
-- Always use the [serial comma](https://en.wikipedia.org/wiki/Serial_comma) in lists.
+- 3つ以上の項目を並べるとき、[最後の「および」の前には読点](https://en.wikipedia.org/wiki/Serial_comma)を打ちます。
-- Avoid referring to the GitHub community as "users."
+- GitHubコミュニティを「ユーザー」と称するのは避けてください。
- **DO:** Create issues automatically for people in your organization
+ **良い例:** Organizationの人に自動的にIssueを作成する
- **DON'T:** Create issues automatically for an organization's users
+ **悪い例:** 組織のユーザーについて自動的にIssueを作成する
-- Avoid acronyms unless they’re well established (such as API).
+- 略語は一般的な場合 (APIなど) を除いて使用しないでください。
-### Product screenshots
+### 製品のスクリーンショット
-You can upload up to five screenshot images of your app to display on your app's landing page. Add an optional caption to each screenshot to provide context. After you upload your screenshots, you can drag them into the order you want them to be displayed on the landing page.
+アプリケーションのランディングページで表示されるように、アプリケーションのスクリーンショット画像を5つまでアップロードできます。 スクリーンショットごとに状況がわかるキャプションをオプションとして追加します。 スクリーンショットをアップロードすると、ランディングページに表示したい順序でドラッグできます。
-#### Guidelines for screenshots
+#### スクリーンショットのガイドライン
-- Images must be of high resolution (at least 1200px wide).
-- All images must be the same height and width (aspect ratio) to avoid page jumps when people click from one image to the next.
-- Show as much of the user interface as possible so people can see what your app does.
-- When taking screenshots of your app in a browser, only include the content in the display window. Avoid including the address bar, title bar, or toolbar icons, which do not scale well to smaller screen sizes.
-- GitHub displays the screenshots you upload in a box on your app's landing page, so you don't need to add boxes or borders around your screenshots.
-- Captions are most effective when they are short and snappy.
+- 画像は高解像度 (幅1200px以上) でなければなりません。
+- 画像を次から次へのクリックしたときにページが移動するのを避けるために、すべての画像は高さと幅 (アスペクト比) を等しくする必要があります。
+- アプリケーションの動作が見えるように、ユーザーインターフェースはできるだけ多く表示してください。
+- ブラウザーでアプリケーションのスクリーンショットを取得するときには、ディスプレイウィンドウの内容のみを含めるようにします。 アドレスバー、タイトルバー、ツールバーのアイコンは含めないでください。小さい画面で適切に縮小されません。
+- アップロードしたスクリーンショットは、アプリケーションのランディングページにあるボックスに表示されるので、スクリーンショットの周囲にボックスや枠線は必要ありません。
+- キャプションは、短く簡潔なほうが効果があります。
-
+
diff --git a/translations/ja-JP/content/developers/overview/about-githubs-apis.md b/translations/ja-JP/content/developers/overview/about-githubs-apis.md
index d72cf182c3e4..b6b267148b5d 100644
--- a/translations/ja-JP/content/developers/overview/about-githubs-apis.md
+++ b/translations/ja-JP/content/developers/overview/about-githubs-apis.md
@@ -1,6 +1,6 @@
---
-title: About GitHub's APIs
-intro: 'Learn about {% data variables.product.prodname_dotcom %}''s APIs to extend and customize your {% data variables.product.prodname_dotcom %} experience.'
+title: GitHub APIについて
+intro: '{% data variables.product.prodname_dotcom %}の体験を拡張し、カスタマイズするために、{% data variables.product.prodname_dotcom %}のAPIについて学んでください。'
redirect_from:
- /v3/versions
versions:
@@ -9,22 +9,22 @@ versions:
github-ae: '*'
---
-There are two stable versions of the GitHub API: the [REST API](/v3/) and the [GraphQL API](/v4/).
+GitHub APIには、[REST API](/v3/)と[GraphQL API](/v4/)という2つの安定バージョンがあります。
-When using the REST API, we encourage you to [request v3 via the `Accept` header](/v3/media/#request-specific-version).
+REST APIを使う際には、[`Accept`ヘッダを介してv3をリクエスト](/v3/media/#request-specific-version)することをおすすめします。
-For information on using the GraphQL API, see the [v4 docs](/v4/).
+GraphQL APIの利用に関する情報については[v4のドキュメント](/v4/)を参照してください。
-## Deprecated versions
+## 非推奨のバージョン
### ベータ
-We deprecated the beta API on April 22, 2014.
+ベータAPIは2014年4月22日に非推奨となりました。
### v2
-We removed support for API v2 on June 12, 2012.
+API v2のサポートは2012年6月12日に廃止されました。
### v1
-We removed support for API v1 on June 12, 2012.
+API v1のサポートは2012年6月12日に廃止されました。
diff --git a/translations/ja-JP/content/developers/overview/github-developer-program.md b/translations/ja-JP/content/developers/overview/github-developer-program.md
index f10a364e70bd..abdd43090026 100644
--- a/translations/ja-JP/content/developers/overview/github-developer-program.md
+++ b/translations/ja-JP/content/developers/overview/github-developer-program.md
@@ -1,37 +1,37 @@
---
-title: GitHub Developer Program
-intro: 'If you build tools that integrate with {% data variables.product.prodname_dotcom %}, you can join the {% data variables.product.prodname_dotcom %} Developer Program.'
+title: GitHub開発者プログラム
+intro: '{% data variables.product.prodname_dotcom %}と統合されるツールを構築するなら、{% data variables.product.prodname_dotcom %}開発者プログラムに参加できます。'
redirect_from:
- - /プログラム
+ - /program
versions:
free-pro-team: '*'
---
-Building an application that integrates with GitHub? Register for our Developer Program! The possibilities are endless, and you enjoy the kudos. [Register now](https://github.com/developer/register)
+GitHubと統合されるアプリケーションを構築しますか? 弊社の開発者プログラムに登録してください! 可能性は無限であり、栄誉を楽しんでください。 [今すぐ登録を](https://github.com/developer/register)
-## Stay in the know
+## 把握し続けてください
-Be the first to know about API changes and try out new features before they launch in the [Developer blog](https://developer.github.com/changes/).
+[開発者ブログ](https://developer.github.com/changes/)でAPIの変更について最初に知り、公開されるより先に新機能を試してください。
-## Scratch an itch
+## かゆいところに手を届ける
-Build your own tools that seamlessly integrate with the place you push code every day.
+毎日コードをプッシュするところとシームレスに統合される独自のツールを構築してください。
-## Take on the enterprise
+## エンタープライズへの挑戦
-[Obtain developer licenses](http://github.com/contact?form%5Bsubject%5D=Development+licenses) to build and test your application against {% data variables.product.prodname_ghe_server %} or {% data variables.product.prodname_ghe_managed %}.
+{% data variables.product.prodname_ghe_server %}あるいは{% data variables.product.prodname_ghe_managed %}に対してアプリケーションを構築してテストするために、[開発者ライセンスを取得](http://github.com/contact?form%5Bsubject%5D=Development+licenses)してください。
-## Have an integration that works with GitHub?
+## GitHubと連携するインテグレーションがありますか?
-Awesome! We'd love to have you be part of the program. Here’s how you can spread the word:
-* [Let us know about your integration](https://github.com/contact?form[subject]=New+GitHub+Integration)
-* Use the [Octocat or GitHub logo](https://github.com/logos) to identify that your product works with GitHub
-* Post a video or a blog on your website about your integration
+素晴らしいです! プログラムに参加していただければ嬉しく思います。 以下のようにすれば、情報を展開できます。
+* [あなたのインテグレーションについてお知らせください。](https://github.com/contact?form[subject]=New+GitHub+Integration)
+* [OctocatもしくはGitHubロゴ](https://github.com/logos)を使って、あなたの製品がGitHubと動作することを示してください。
+* Webサイトにインテグレーションに関するビデオあるいはブログをポストしてください。
-## Ready to join the GitHub Developer Program?
+## GitHub開発者プログラムに参加する準備はできましたか?
-Membership is open to individual developers and companies who have:
+メンバーシップは、以下をお持ちの個人開発者及び企業に対して開かれています。
-* An integration in production or development using the GitHub API.
-* An email address where GitHub users can contact you for support.
+* GitHub APIを使用した、実働中あるいは開発中のインテグレーション。
+* GitHubユーザがサポートのために連絡できるメールアドレス。
diff --git a/translations/ja-JP/content/developers/overview/index.md b/translations/ja-JP/content/developers/overview/index.md
index e0741f828de0..132fb50afca5 100644
--- a/translations/ja-JP/content/developers/overview/index.md
+++ b/translations/ja-JP/content/developers/overview/index.md
@@ -1,6 +1,6 @@
---
title: 概要
-intro: 'Learn about {% data variables.product.prodname_dotcom %}''s APIs{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %} and secure your deployments.{% else %}, secure your deployments, and join {% data variables.product.prodname_dotcom %}''s Developer Program.{% endif %}'
+intro: '{% data variables.product.prodname_dotcom %}のAPIに付いて学び、{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}デプロイメントをセキュアにしてください。{% else %}デプロイメントをセキュアにし、{% data variables.product.prodname_dotcom %}の開発者プログラムに参加してください。{% endif %}'
versions:
free-pro-team: '*'
enterprise-server: '*'
diff --git a/translations/ja-JP/content/developers/overview/managing-deploy-keys.md b/translations/ja-JP/content/developers/overview/managing-deploy-keys.md
index 28b333458057..250482473af4 100644
--- a/translations/ja-JP/content/developers/overview/managing-deploy-keys.md
+++ b/translations/ja-JP/content/developers/overview/managing-deploy-keys.md
@@ -1,6 +1,6 @@
---
-title: Managing deploy keys
-intro: Learn different ways to manage SSH keys on your servers when you automate deployment scripts and which way is best for you.
+title: デプロイキーの管理
+intro: デプロイメントのスクリプトを自動化する際にサーバー上のSSHキーを管理する様々な方法と、どれが最適な方法かを学んでください。
redirect_from:
- /guides/managing-deploy-keys/
- /v3/guides/managing-deploy-keys
@@ -11,48 +11,48 @@ versions:
---
-You can manage SSH keys on your servers when automating deployment scripts using SSH agent forwarding, HTTPS with OAuth tokens, deploy keys, or machine users.
+SSHエージェントのフォワーディング、OAuthトークンでのHTTPS、デプロイキー、マシンユーザを使ってデプロイメントスクリプトを自動化する際に、サーバー上のSSHキーを管理できます。
-### SSH agent forwarding
+### SSHエージェントのフォワーディング
-In many cases, especially in the beginning of a project, SSH agent forwarding is the quickest and simplest method to use. Agent forwarding uses the same SSH keys that your local development computer uses.
+多くの場合、特にプロジェクトの開始時には、SSHエージェントのフォワーディングが最も素早くシンプルに使える方法です。 エージェントのフォワーディングでは、ローカルの開発コンピュータで使うのと同じSSHキーを使います。
-##### Pros
+##### 長所
-* You do not have to generate or keep track of any new keys.
-* There is no key management; users have the same permissions on the server that they do locally.
-* No keys are stored on the server, so in case the server is compromised, you don't need to hunt down and remove the compromised keys.
+* 新しいキーを生成したり追跡したりしなくていい。
+* キーの管理は不要。ユーザはローカルと同じ権限をサーバーでも持つ。
+* サーバーにキーは保存されないので、サーバーが侵害を受けた場合でも、侵害されたキーを追跡して削除する必要はない。
-##### Cons
+##### 短所
-* Users **must** SSH in to deploy; automated deploy processes can't be used.
-* SSH agent forwarding can be troublesome to run for Windows users.
+* ユーザはデプロイするためにSSH**しなければならない**。自動化されたデプロイプロセスは利用できない。
+* SSHエージェントのフォワーディングは、Windowsのユーザが実行するのが面倒。
##### セットアップ
-1. Turn on agent forwarding locally. See [our guide on SSH agent forwarding][ssh-agent-forwarding] for more information.
-2. Set your deploy scripts to use agent forwarding. For example, on a bash script, enabling agent forwarding would look something like this: `ssh -A serverA 'bash -s' < deploy.sh`
+1. エージェントのフォワーディングをローカルでオンにしてください。 詳しい情報については[SSHエージェントフォワーディングのガイド][ssh-agent-forwarding]を参照してください。
+2. エージェントフォワーディングを使用するように、デプロイスクリプトを設定してください。 たとえばbashのスクリプトでは、以下のようにしてエージェントのフォワーディングを有効化することになるでしょう。 `ssh -A serverA 'bash -s' < deploy.sh`
-### HTTPS cloning with OAuth tokens
+### OAuthトークンを使ったHTTPSでのクローニング
-If you don't want to use SSH keys, you can use [HTTPS with OAuth tokens][git-automation].
+SSHキーを使いたくないなら、[OAuthトークンでHTTPS][git-automation]を利用できます。
-##### Pros
+##### 長所
-* Anyone with access to the server can deploy the repository.
-* Users don't have to change their local SSH settings.
-* Multiple tokens (one for each user) are not needed; one token per server is enough.
-* A token can be revoked at any time, turning it essentially into a one-use password.
-* Generating new tokens can be easily scripted using [the OAuth API](/rest/reference/oauth-authorizations#create-a-new-authorization).
+* サーバーにアクセスできる人なら、リポジトリをデプロイできる。
+* ユーザはローカルのSSH設定を変更する必要がない。
+* 複数のトークン(ユーザごと)が必要ない。サーバーごとに1つのトークンで十分。
+* トークンはいつでも取り消しできるので、本質的には使い捨てのパスワードにすることができる。
+* 新しいトークンの作成は、[OAuth API](/rest/reference/oauth-authorizations#create-a-new-authorization)を使って容易にスクリプト化できる。
-##### Cons
+##### 短所
-* You must make sure that you configure your token with the correct access scopes.
-* Tokens are essentially passwords, and must be protected the same way.
+* トークンを確実に正しいアクセススコープで設定しなければならない。
+* トークンは本質的にはパスワードであり、パスワードと同じように保護しなければならない。
##### セットアップ
-See [our guide on Git automation with tokens][git-automation].
+[トークンでのGit自動化ガイド][git-automation]を参照してください。
### デプロイキー
@@ -60,33 +60,33 @@ See [our guide on Git automation with tokens][git-automation].
{% data reusables.repositories.deploy-keys-write-access %}
-##### Pros
+##### 長所
-* Anyone with access to the repository and server has the ability to deploy the project.
-* Users don't have to change their local SSH settings.
-* Deploy keys are read-only by default, but you can give them write access when adding them to a repository.
+* リポジトリとサーバーにアクセスできる人は、誰でもプロジェクトをデプロイできる。
+* ユーザはローカルのSSH設定を変更する必要がない。
+* デプロイキーはデフォルトではリードオンリーだが、リポジトリに追加する際には書き込みアクセス権を与えることができる。
-##### Cons
+##### 短所
-* Deploy keys only grant access to a single repository. More complex projects may have many repositories to pull to the same server.
-* Deploy keys are usually not protected by a passphrase, making the key easily accessible if the server is compromised.
+* デプロイキーは単一のリポジトリに対するアクセスだけを許可できる。 より複雑なプロジェクトは、同じサーバーからプルする多くのリポジトリを持っていることがある。
+* デプロイキーは通常パスフレーズで保護されていないので、サーバーが侵害されると簡単にキーにアクセスされることになる。
##### セットアップ
-1. [Run the `ssh-keygen` procedure][generating-ssh-keys] on your server, and remember where you save the generated public/private rsa key pair.
-2. In the upper-right corner of any {% data variables.product.product_name %} page, click your profile photo, then click **Your profile**. 
-3. On your profile page, click **Repositories**, then click the name of your repository. 
-4. From your repository, click **Settings**. 
-5. In the sidebar, click **Deploy Keys**, then click **Add deploy key**. 
-6. Provide a title, paste in your public key. 
-7. Select **Allow write access** if you want this key to have write access to the repository. A deploy key with write access lets a deployment push to the repository.
-8. Click **Add key**.
+1. サーバー上で[`ssh-keygen`の手順を実行][generating-ssh-keys]し、生成された公開/秘密RSAキーのペアを保存した場所を覚えておいてください。
+2. {% data variables.product.product_name %}の任意のページの右上で、プロフィールの写真をクリックし、続いて**Your profile(あなたのプロフィール)**をクリックしてください。 
+3. プロフィールページで**Repositories(リポジトリ)**をクリックし、続いてリポジトリの名前をクリックしてください。 
+4. リポジトリで**Settings(設定)**をクリックしてください。 
+5. サイドバーで**Deploy Keys(デプロイキー)**をクリックし、続いて**Add deploy key(デプロイキーの追加)**をクリックしてください。 
+6. タイトルを入力し、公開鍵に貼り付けてください。 
+7. このキーにリポジトリへの書き込みアクセスを許可したい場合は、**Allow write access(書き込みアクセスの許可)**を選択してください。 書き込みアクセス権を持つデプロイキーを使うと、リポジトリにデプロイメントのプッシュができるようになります。
+8. **Add key(キーの追加)**をクリックしてください。
-##### Using multiple repositories on one server
+##### 1つのサーバー上で複数のリポジトリを利用する
-If you use multiple repositories on one server, you will need to generate a dedicated key pair for each one. You can't reuse a deploy key for multiple repositories.
+1つのサーバー上で複数のリポジトリを使うなら、それぞれのリポジトリに対して専用のキーペアを生成しなければなりません。 複数のリポジトリでデプロイキーを再利用することはできません。
-In the server's SSH configuration file (usually `~/.ssh/config`), add an alias entry for each repository. 例:
+サーバーのSSH設定ファイル(通常は`~/.ssh/config`)に、それぞれのリポジトリに対してエイリアスエントリを追加してください。 例:
```bash
Host {% if currentVersion == "free-pro-team@latest" %}github.com{% else %}my-GHE-hostname.com{% endif %}-repo-0
@@ -98,49 +98,49 @@ Host {% if currentVersion == "free-pro-team@latest" %}github.com{% else %}my-GHE
IdentityFile=/home/user/.ssh/repo-1_deploy_key
```
-* `Host {% if currentVersion == "free-pro-team@latest" %}github.com{% else %}my-GHE-hostname.com{% endif %}-repo-0` - The repository's alias.
-* `Hostname {% if currentVersion == "free-pro-team@latest" %}github.com{% else %}my-GHE-hostname.com{% endif %}` - Configures the hostname to use with the alias.
-* `IdentityFile=/home/user/.ssh/repo-0_deploy_key` - Assigns a private key to the alias.
+* `Host {% if currentVersion == "free-pro-team@latest" %}github.com{% else %}my-GHE-hostname.com{% endif %}-repo-0` - リポジトリのエイリアス。
+* `Hostname {% if currentVersion == "free-pro-team@latest" %}github.com{% else %}my-GHE-hostname.com{% endif %}` - エイリアスで使うホスト名の設定。
+* `IdentityFile=/home/user/.ssh/repo-0_deploy_key` - このエイリアスに秘密鍵を割り当てる。
-You can then use the hostname's alias to interact with the repository using SSH, which will use the unique deploy key assigned to that alias. 例:
+こうすれば、ホスト名のエイリアスを使ってSSHでリポジトリとやりとりできます。この場合、このエイリアスに割り当てられたユニークなデプロイキーが使われます。 例:
```bash
$ git clone git@{% if currentVersion == "free-pro-team@latest" %}github.com{% else %}my-GHE-hostname.com{% endif %}-repo-1:OWNER/repo-1.git
```
-### Machine users
+### マシンユーザ
-If your server needs to access multiple repositories, you can create a new {% data variables.product.product_name %} account and attach an SSH key that will be used exclusively for automation. Since this {% data variables.product.product_name %} account won't be used by a human, it's called a _machine user_. You can add the machine user as a [collaborator][collaborator] on a personal repository (granting read and write access), as an [outside collaborator][outside-collaborator] on an organization repository (granting read, write, or admin access), or to a [team][team] with access to the repositories it needs to automate (granting the permissions of the team).
+サーバーが複数のリポジトリにアクセスしなければならないのであれば、自動化専用に使われる新しい{% data variables.product.product_name %}アカウントを作成し、SSHキーを添付できます。 この{% data variables.product.product_name %}アカウントは人によって使われることはないので、_マシンユーザ_と呼ばれます。 マシンユーザは、個人リポジトリには[コラボレータ][collaborator]として(読み書きのアクセスを許可)、Organizationのリポジトリには[外部のコラボレータ][outside-collaborator]として(読み書き及び管理アクセスを許可)、あるいは自動化する必要があるリポジトリへのアクセスを持つ[Team][team]に(そのTeamの権限を許可)追加できます。
{% if currentVersion == "free-pro-team@latest" %}
{% tip %}
-**Tip:** Our [terms of service][tos] state:
+**Tip:** [利用規約][tos]では以下のように述べられています。
> *「ボット」またはその他の自動化された手段で「アカウント」を登録することは許可されていません。*
-This means that you cannot automate the creation of accounts. But if you want to create a single machine user for automating tasks such as deploy scripts in your project or organization, that is totally cool.
+これは、アカウントの生成を自動化することはできないということです。 しかし、プロジェクトやOrganization内でデプロイスクリプトのような自動化タスクのために1つのマシンユーザを作成したいなら、それはまったく素晴らしいことです。
{% endtip %}
{% endif %}
-##### Pros
+##### 長所
-* Anyone with access to the repository and server has the ability to deploy the project.
-* No (human) users need to change their local SSH settings.
-* Multiple keys are not needed; one per server is adequate.
+* リポジトリとサーバーにアクセスできる人は、誰でもプロジェクトをデプロイできる。
+* (人間の)ユーザがローカルのSSH設定を変更する必要がない。
+* 複数のキーは必要ない。サーバーごとに1つでよい。
-##### Cons
+##### 短所
-* Only organizations can restrict machine users to read-only access. Personal repositories always grant collaborators read/write access.
-* Machine user keys, like deploy keys, are usually not protected by a passphrase.
+* Organizationだけがマシンユーザをリードオンリーのアクセスにできる。 個人リポジトリは、常にコラボレータの読み書きアクセスを許可する。
+* マシンユーザのキーは、デプロイキーのように、通常パスフレーズで保護されない。
##### セットアップ
-1. [Run the `ssh-keygen` procedure][generating-ssh-keys] on your server and attach the public key to the machine user account.
-2. Give the machine user account access to the repositories you want to automate. You can do this by adding the account as a [collaborator][collaborator], as an [outside collaborator][outside-collaborator], or to a [team][team] in an organization.
+1. サーバー上で[`ssh-keygen`の手順を実行][generating-ssh-keys]し、公開鍵をマシンユーザアカウントに添付してください。
+2. マシンユーザアカウントに自動化したいリポジトリへのアクセスを付与してください。 これは、アカウントを[コラボレータ][collaborator]、[外部のコラボレータ][outside-collaborator]として、あるいはOrganization内の[Team][team]に追加することでも行えます。
[ssh-agent-forwarding]: /guides/using-ssh-agent-forwarding/
[generating-ssh-keys]: /articles/generating-a-new-ssh-key-and-adding-it-to-the-ssh-agent/#generating-a-new-ssh-key
diff --git a/translations/ja-JP/content/developers/overview/replacing-github-services.md b/translations/ja-JP/content/developers/overview/replacing-github-services.md
index 878a316bd5b2..e82ee2397e8f 100644
--- a/translations/ja-JP/content/developers/overview/replacing-github-services.md
+++ b/translations/ja-JP/content/developers/overview/replacing-github-services.md
@@ -1,6 +1,6 @@
---
-title: Replacing GitHub Services
-intro: 'If you''re still relying on the deprecated {% data variables.product.prodname_dotcom %} Services, learn how to migrate your service hooks to webhooks.'
+title: GitHub Servicesの置き換え
+intro: '非推奨となった{% data variables.product.prodname_dotcom %} Servicesにまだ依存しているなら、サービスフックをwebhookに移行する方法を学んでください。'
redirect_from:
- /guides/replacing-github-services/
- /v3/guides/automating-deployments-to-integrators/
@@ -11,61 +11,61 @@ versions:
---
-We have deprecated GitHub Services in favor of integrating with webhooks. This guide helps you transition to webhooks from GitHub Services. For more information on this announcement, see the [blog post](https://developer.github.com/changes/2018-10-01-denying-new-github-services).
+GitHub Servicesは、webhookとの統合を進めるために非推奨となりました。 このガイドは、GitHub Servicesからwebhookへの移行を支援します。 このアナウンスに関する詳細については、[ブログポスト](https://developer.github.com/changes/2018-10-01-denying-new-github-services)を参照してください。
{% note %}
-As an alternative to the email service, you can now start using email notifications for pushes to your repository. See "[About email notifications for pushes to your repository](/github/receiving-notifications-about-activity-on-github/about-email-notifications-for-pushes-to-your-repository/)" to learn how to configure commit email notifications.
+メールサービスに代わるものとして、リポジトリへのプッシュに対するメール通知を利用しはじめられるようになりました。 コミットメール通知の設定方法については、「[リポジトリへのプッシュに対するメール通知について](/github/receiving-notifications-about-activity-on-github/about-email-notifications-for-pushes-to-your-repository/)」を参照してください。
{% endnote %}
-### Deprecation timeline
+### 非推奨のタイムライン
-- **October 1, 2018**: GitHub discontinued allowing users to install services. We removed GitHub Services from the GitHub.com user interface.
-- **January 29, 2019**: As an alternative to the email service, you can now start using email notifications for pushes to your repository. See "[About email notifications for pushes to your repository](/github/receiving-notifications-about-activity-on-github/about-email-notifications-for-pushes-to-your-repository/)" to learn how to configure commit email notifications.
-- **January 31, 2019**: GitHub will stop delivering installed services' events on GitHub.com.
+- **2018年10月1日**: GitHubはユーザがサービスをインストールするのを禁止しました。 GitHub.comのユーザインターフェースから、GitHub Servicesを削除しました。
+- **2019年1月29日**: メールサービスの代替として、リポジトリへのプッシュに対するメール通知を使い始められるようになりました。 コミットメール通知の設定方法については、「[リポジトリへのプッシュに対するメール通知について](/github/receiving-notifications-about-activity-on-github/about-email-notifications-for-pushes-to-your-repository/)」を参照してください。
+- **2019年1月31日**: GitHubはGitHub.com上でのインストールされたサービスのイベント配信を停止しました。
-### GitHub Services background
+### GitHub Servicesの背景
-GitHub Services (sometimes referred to as Service Hooks) is the legacy method of integrating where GitHub hosted a portion of our integrator’s services via [the `github-services` repository](https://github.com/github/github-services). Actions performed on GitHub trigger these services, and you can use these services to trigger actions outside of GitHub.
+GitHub Services(Service Hooksと呼ばれることもあります)は、インテグレーションの旧来の方法であり、GitHubがインテグレーターのサービスの一部を[`github-services`リポジトリ](https://github.com/github/github-services)を通じてホストします。 GitHub上で行われたアクションがこれらのサービスをトリガーし、これらのサービスを使ってGitHubの外部のアクションをトリガーできます。
{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-### Finding repositories that use GitHub Services
-We provide a command-line script that helps you identify which repositories on your appliance use GitHub Services. For more information, see [ghe-legacy-github-services-report](/enterprise/{{currentVersion}}/admin/articles/command-line-utilities/#ghe-legacy-github-services-report).{% endif %}
+### GitHub Servicesを使っているリポジトリを探す
+アプライアンス上でどのリポジトリがGitHub Servicesを使っているかを特定するためのコマンドラインスクリプトが提供されています。 詳しい情報については[ghe-legacy-github-services-report](/enterprise/{{currentVersion}}/admin/articles/command-line-utilities/#ghe-legacy-github-services-report)を参照してください。{% endif %}
-### GitHub Services vs. webhooks
+### GitHub Servicesとwebhook
-The key differences between GitHub Services and webhooks:
-- **Configuration**: GitHub Services have service-specific configuration options, while webhooks are simply configured by specifying a URL and a set of events.
-- **Custom logic**: GitHub Services can have custom logic to respond with multiple actions as part of processing a single event, while webhooks have no custom logic.
-- **Types of requests**: GitHub Services can make HTTP and non-HTTP requests, while webhooks can make HTTP requests only.
+GitHub Servicesとwebhookとの主な違いは以下のとおりです。
+- **設定**: GitHub Servicesにはサービス固有の設定オプションがありますが、webhookはURLとイベント群を指定するだけで単純に設定できます。
+- **カスタムロジック**: GitHub Servicesは1つのイベントの処理の一部として、複数のアクションで反応するカスタムロジックを持つことができますが、webhookにはカスタムロジックはありません。
+- **リクエストの種類**: GitHub ServicesはHTTP及び非HTTPリクエストを発行できますが、webhookが発行できるのはHTTPリクエストのみです。
-### Replacing Services with webhooks
+### webhookでのServicesの置き換え
-To replace GitHub Services with Webhooks:
+GitHub Servicesをwebhookで置き換えるには、以下のようにします。
-1. Identify the relevant webhook events you’ll need to subscribe to from [this list](/webhooks/#events).
+1. [このリスト](/webhooks/#events)から、サブスクライブする必要がある関連webhookイベントを特定してください。
-2. Change your configuration depending on how you currently use GitHub Services:
+2. GitHub Servicesを現在使っている方法に応じて、設定を変更してください。
- - **GitHub Apps**: Update your app's permissions and subscribed events to configure your app to receive the relevant webhook events.
- - **OAuth Apps**: Request either the `repo_hook` and/or `org_hook` scope(s) to manage the relevant events on behalf of users.
- - **GitHub Service providers**: Request that users manually configure a webhook with the relevant events sent to you, or take this opportunity to build an app to manage this functionality. For more information, see "[About apps](/apps/about-apps/)."
+ - **GitHub Apps**: アプリケーションの権限とサブスクライブしているイベントを更新し、関連するwebhookイベントを受信するようにアプリケーションを設定してください。
+ - **OAuth Apps**: `repo_hook`や`org_hook`スコープをリクエストして、ユーザの代わりに関連するイベントを管理してください。
+ - **GitHub Serviceプロバイダー**: ユーザが手動で、送信された関連するイベントとあわせてwebhookを設定するように要求するか、この機会にこの機能を管理するアプリケーションを構築してください。 詳しい情報については「[アプリケーションについて](/apps/about-apps/)」を参照してください。
-3. Move additional configuration from outside of GitHub. Some GitHub Services require additional, custom configuration on the configuration page within GitHub. If your service does this, you will need to move this functionality into your application or rely on GitHub or OAuth Apps where applicable.
+3. GitHubの外部から、追加の設定を移動してください。 GitHub Servicesの中には、GitHub内の設定ページで追加のカスタム設定が必要になるものがあります。 使っているサービスがそうなら、この機能をアプリケーションに移すか、可能な場合はGitHub AppもしくはOAuth Appに依存する必要があります。
-### Supporting {% data variables.product.prodname_ghe_server %}
+### {% data variables.product.prodname_ghe_server %}のサポート
-- **{% data variables.product.prodname_ghe_server %} 2.17**: {% data variables.product.prodname_ghe_server %} release 2.17 and higher will discontinue allowing admins to install services. Admins will continue to be able to modify existing service hooks and receive service hooks in {% data variables.product.prodname_ghe_server %} release 2.17 through 2.19. As an alternative to the email service, you will be able to use email notifications for pushes to your repository in {% data variables.product.prodname_ghe_server %} 2.17 and higher. See [this blog post](https://developer.github.com/changes/2019-01-29-life-after-github-services) to learn more.
-- **{% data variables.product.prodname_ghe_server %} 2.20**: {% data variables.product.prodname_ghe_server %} release 2.20 and higher will stop delivering all installed services' events.
+- **{% data variables.product.prodname_ghe_server %} 2.17**: {% data variables.product.prodname_ghe_server %} リリース2.17以降では、管理者がサービスをインストールできなくなります。 {% data variables.product.prodname_ghe_server %}リリース2.17から2.19では、管理者は引き続き既存のサービスフックを変更し、サービスフックを受信できます。 {% data variables.product.prodname_ghe_server %} 2.17以降では、メールサービスの代替としてリポジトリへのプッシュに対するメール通知が使えます。 詳細については[このブログポスト](https://developer.github.com/changes/2019-01-29-life-after-github-services)を参照してください。
+- **{% data variables.product.prodname_ghe_server %} 2.20**: {% data variables.product.prodname_ghe_server %}リリース2.20以降では、インストールされたすべてのサービスイベントの配信が停止されます。
-The {% data variables.product.prodname_ghe_server %} 2.17 release will be the first release that does not allow admins to install GitHub Services. We will only support existing GitHub Services until the {% data variables.product.prodname_ghe_server %} 2.20 release. We will also accept any critical patches for your GitHub Service running on {% data variables.product.prodname_ghe_server %} until October 1, 2019.
+{% data variables.product.prodname_ghe_server %} 2.17リリースは、管理者がGitHub Servicesをインストールできない最初のリリースになります。 既存のGitHub Servicesは、{% data variables.product.prodname_ghe_server %} 2.20リリースまでしかサポートされません。 また、2019年10月1日まで{% data variables.product.prodname_ghe_server %}上で動作しているGitHub Serviceに対する重要なパッチを受け付けます。
-### Migrating with our help
+### 弊社の支援を受けての移行
-Please [contact us](https://github.com/contact?form%5Bsubject%5D=GitHub+Services+Deprecation) with any questions.
+質問があれば、[お問い合わせ](https://github.com/contact?form%5Bsubject%5D=GitHub+Services+Deprecation)ください。
-As a high-level overview, the process of migration typically involves:
- - Identifying how and where your product is using GitHub Services.
- - Identifying the corresponding webhook events you need to configure in order to move to plain webhooks.
- - Implementing the design using either [{% data variables.product.prodname_oauth_app %}s](/apps/building-oauth-apps/) or [{% data variables.product.prodname_github_app %}s. {% data variables.product.prodname_github_app %}s](/apps/building-github-apps/) are preferred. To learn more about why {% data variables.product.prodname_github_app %}s are preferred, see "[Reasons for switching to {% data variables.product.prodname_github_app %}s](/apps/migrating-oauth-apps-to-github-apps/#reasons-for-switching-to-github-apps)."
+高レベルの概要としては、移行のプロセスは通常以下を含みます。
+ - 製品がどこでどのようにGitHub Servicesを使っているかの特定。
+ - 通常のwebhookに移行するために設定する必要がある、対応するwebhookイベントの特定。
+ - [{% data variables.product.prodname_oauth_app %}](/apps/building-oauth-apps/)もしくは[{% data variables.product.prodname_github_app %}](/apps/building-github-apps/)のいずれかを利用して設計を実装。 {% data variables.product.prodname_github_app %}の方が望ましいです。 {% data variables.product.prodname_github_app %}が望ましい理由の詳細を学ぶには、「[{% data variables.product.prodname_github_app %}に切り替える理由](/apps/migrating-oauth-apps-to-github-apps/#reasons-for-switching-to-github-apps)」を参照してください。
diff --git a/translations/ja-JP/content/developers/overview/secret-scanning.md b/translations/ja-JP/content/developers/overview/secret-scanning.md
index bf0a60000a02..66751d7e4136 100644
--- a/translations/ja-JP/content/developers/overview/secret-scanning.md
+++ b/translations/ja-JP/content/developers/overview/secret-scanning.md
@@ -1,6 +1,6 @@
---
title: Secret scanning
-intro: 'As a service provider, you can partner with {% data variables.product.prodname_dotcom %} to have your secret token formats secured through secret scanning, which searches for accidental commits of your secret format and can be sent to a service provider''s verify endpoint.'
+intro: 'サービスプロバイダーは、{% data variables.product.prodname_dotcom %}とパートナーになり、シークレットスキャンニングを通じてシークレットトークンのフォーマットを保護できます。シークレットスキャンニングは、そのシークレットのフォーマットで誤って行われたコミットを検索し、サービスプロバイダーの検証用エンドポイントに送信します。'
redirect_from:
- /partnerships/token-scanning/
- /partnerships/secret-scanning
@@ -9,39 +9,39 @@ versions:
---
-{% data variables.product.prodname_dotcom %} scans repositories for known secret formats to prevent fraudulent use of credentials that were committed accidentally. Secret scanning happens by default on public repositories, and can be enabled on private repositories by repository administrators or organization owners. As a service provider, you can partner with {% data variables.product.prodname_dotcom %} so that your secret formats are included in our secret scanning.
+{% data variables.product.prodname_dotcom %}は、既知のシークレットフォーマットに対してリポジトリをスキャンし、誤ってコミットされたクレデンシャルが不正利用されることを防ぎます。 シークレットスキャンニングは、デフォルトでパブリックなリポジトリで行われ、プライベートリポジトリではリポジトリ管理者もしくはOrganizationのオーナーが有効化できます。 サービスプロバイダーは{% data variables.product.prodname_dotcom %}とパートナーになり、シークレットのフォーマットがシークレットスキャンニングに含まれるようにすることができます。
-When a match of your secret format is found in a public repository, a payload is sent to an HTTP endpoint of your choice.
+シークレットのフォーマットに対する一致がパブリックリポジトリで見つかった場合、選択したHTTPのエンドポイントにペイロードが送信されます。
-When a match of your secret format is found in a private repository configured for secret scanning, then repository admins are alerted and can view and manage the secret scanning results on {% data variables.product.prodname_dotcom %}. For more information, see "[Managing alerts from secret scanning](/github/administering-a-repository/managing-alerts-from-secret-scanning)".
+シークレットスキャンニングが設定されたプライベートリポジトリでシークレットフォーマットへの一致が見つかった場合、リポジトリの管理者にはアラートが発せられ、{% data variables.product.prodname_dotcom %}上でシークレットスキャンニングの結果を見て管理できます。 詳しい情報については「[シークレットスキャンニングからのアラートの管理](/github/administering-a-repository/managing-alerts-from-secret-scanning)」を参照してください。
{% note %}
-**Note:** Secret scanning for private repositories is currently in beta.
+**ノート:** プライベートリポジトリに対するシークレットスキャンニングは、現在ベータです。
{% endnote %}
-This article describes how you can partner with {% data variables.product.prodname_dotcom %} as a service provider and join the secret scanning program.
+この記事では、サービスプロバイダーとして{% data variables.product.prodname_dotcom %}とパートナーになり、シークレットスキャンニングプログラムに参加する方法を説明します。
-### The secret scanning process
+### シークレットスキャンニングのプロセス
-##### How secret scanning works in a public repository
+##### パブリックリポジトリでのシークレットスキャンニングの動作
-The following diagram summarizes the secret scanning process for public repositories, with any matches sent to a service provider's verify endpoint.
+以下の図は、パブリックリポジトリに対するシークレットスキャンニングのプロセスをまとめたもので、一致があった場合にサービスプロバイダへの検証エンドポイントに送信されています。

-### Joining the secret scanning program on {% data variables.product.prodname_dotcom %}
+### {% data variables.product.prodname_dotcom %}上のシークレットスキャンニングプログラムへの参加
-1. Contact {% data variables.product.prodname_dotcom %} to get the process started.
-1. Identify the relevant secrets you want to scan for and create regular expressions to capture them.
-1. For secret matches found in public repositories, create a secret alert service which accepts webhooks from {% data variables.product.prodname_dotcom %} that contain the secret scanning message payload.
-1. Implement signature verification in your secret alert service.
-1. Implement secret revocation and user notification in your secret alert service.
+1. プロセスを開始するために、{% data variables.product.prodname_dotcom %}に連絡してください。
+1. スキャンしたい関連シークレットを特定し、それらを捕捉するための正規表現を作成してください。
+1. パブリックリポジトリで見つかったシークレットの一致に対応するために、シークレットスキャンニングのメッセージペイロードを含む{% data variables.product.prodname_dotcom %}からのwebhookを受け付けるシークレットアラートサービスを作成してください。
+1. シークレットアラートサービスに、署名検証を実装してください。
+1. シークレットアラートサービスに、シークレットの破棄とユーザへの通知を実装してください。
-#### Contact {% data variables.product.prodname_dotcom %} to get the process started
+#### プロセスを開始するための{% data variables.product.prodname_dotcom %}への連絡
-To get the enrollment process started, email secret-scanning@github.com.
+登録のプロセスを開始するために、secret-scanning@github.comにメールしてください。
You will receive details on the secret scanning program, and you will need to agree to {% data variables.product.prodname_dotcom %}'s terms of participation before proceeding.
diff --git a/translations/ja-JP/content/developers/webhooks-and-events/webhook-events-and-payloads.md b/translations/ja-JP/content/developers/webhooks-and-events/webhook-events-and-payloads.md
index 9028359cd6ce..c37e469883d3 100644
--- a/translations/ja-JP/content/developers/webhooks-and-events/webhook-events-and-payloads.md
+++ b/translations/ja-JP/content/developers/webhooks-and-events/webhook-events-and-payloads.md
@@ -1288,7 +1288,7 @@ The event’s actor is the [user](/v3/users/) who starred a repository, and the
{{ webhookPayloadsForCurrentVersion.watch.started }}
-{% if currentVersion == "free-pro-team@latest" %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
### workflow_dispatch
This event occurs when someone triggers a workflow run on GitHub or sends a `POST` request to the "[Create a workflow dispatch event](/rest/reference/actions/#create-a-workflow-dispatch-event)" endpoint. 詳しい情報については、「[ワークフローをトリガーするイベント](/actions/reference/events-that-trigger-workflows#workflow_dispatch)」を参照してください。
@@ -1302,9 +1302,10 @@ This event occurs when someone triggers a workflow run on GitHub or sends a `POS
{{ webhookPayloadsForCurrentVersion.workflow_dispatch }}
{% endif %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %}
### workflow_run
-When a {% data variables.product.prodname_actions %} workflow run is requested or completed. 詳しい情報については、「[ワークフローをトリガーするイベント](/actions/reference/events-that-trigger-workflows#workflow_run)」を参照してください。
+When a {% data variables.product.prodname_actions %} workflow run is requested or completed. For more information, see "[Events that trigger workflows](/actions/reference/events-that-trigger-workflows#workflow_run)."
#### Availability
@@ -1322,3 +1323,4 @@ When a {% data variables.product.prodname_actions %} workflow run is requested o
#### Webhook payload example
{{ webhookPayloadsForCurrentVersion.workflow_run }}
+{% endif %}
diff --git a/translations/ja-JP/content/github/administering-a-repository/about-secret-scanning.md b/translations/ja-JP/content/github/administering-a-repository/about-secret-scanning.md
index 95f5032fb513..54820b441cf1 100644
--- a/translations/ja-JP/content/github/administering-a-repository/about-secret-scanning.md
+++ b/translations/ja-JP/content/github/administering-a-repository/about-secret-scanning.md
@@ -18,6 +18,8 @@ versions:
### パブリックリポジトリの {% data variables.product.prodname_secret_scanning %} について
+ {% data variables.product.prodname_secret_scanning_caps %} is automatically enabled on public repositories, where it scans code for secrets, to check for known secret formats. When a match of your secret format is found in a public repository, {% data variables.product.company_short %} doesn't publicly disclose the information as an alert, but instead sends a payload to an HTTP endpoint of your choice. For an overview of how secret scanning works on public repositories, see "[Secret scanning](/developers/overview/secret-scanning)."
+
パブリックリポジトリにプッシュすると、{% data variables.product.product_name %} がコミットの内容をスキャンしてシークレットを探します。 プライベートリポジトリをパブリックに切り替えると、{% data variables.product.product_name %} はリポジトリ全体をスキャンしてシークレットを探します。
{% data variables.product.prodname_secret_scanning %} が認証情報一式を検出すると、弊社はそのシークレットを発行したサービスプロバイダに通知します。 サービスプロバイダは認証情報を検証し、シークレットを取り消すか、新しいシークレットを発行するか、または直接連絡する必要があるかを決定します。これは、ユーザまたはサービスプロバイダに関連するリスクに依存します。
@@ -65,6 +67,8 @@ versions:
{% data reusables.secret-scanning.beta %}
+If you're a repository administrator or an organization owner, you can enable {% data variables.product.prodname_secret_scanning %} for private repositories that are owned by organizations. You can enable {% data variables.product.prodname_secret_scanning %} for all your repositories, or for all new repositories within your organization. {% data variables.product.prodname_secret_scanning_caps %} is not available for user account-owned private repositories. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository)" and "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)."
+
{% data variables.product.prodname_secret_scanning %} が有効化されているプライベートリポジトリにコミットをプッシュすると、{% data variables.product.product_name %} はコミットの内容をスキャンしてシークレットを探します。
{% data variables.product.prodname_secret_scanning %} がプライベートリポジトリでシークレットを検出すると、{% data variables.product.prodname_dotcom %} はアラートを送信します。
@@ -73,6 +77,8 @@ versions:
- {% data variables.product.prodname_dotcom %} は、リポジトリにアラートを表示します。 詳しい情報については、「[{% data variables.product.prodname_secret_scanning %} からのアラートを管理する](/github/administering-a-repository/managing-alerts-from-secret-scanning)」を参照してください。
+Repository administrators and organization owners can grant users and team access to {% data variables.product.prodname_secret_scanning %} alerts. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-security-alerts)."
+
現在 {% data variables.product.product_name %} は、プライベートリポジトリをスキャンして、次のサービスプロバイダが発行したシークレットを探します。
- Adafruit
diff --git a/translations/ja-JP/content/github/administering-a-repository/classifying-your-repository-with-topics.md b/translations/ja-JP/content/github/administering-a-repository/classifying-your-repository-with-topics.md
index ae066519af19..6d43dcb98224 100644
--- a/translations/ja-JP/content/github/administering-a-repository/classifying-your-repository-with-topics.md
+++ b/translations/ja-JP/content/github/administering-a-repository/classifying-your-repository-with-topics.md
@@ -22,7 +22,7 @@ Topics を利用すれば、特定の領域に関するリポジトリを調べ
リポジトリの管理者は、リポジトリに好きなトピックを追加できます。 Helpful topics to classify a repository include the repository's intended purpose, subject area, community, or language.{% if currentVersion == "free-pro-team@latest" %} Additionally, {% data variables.product.product_name %} analyzes public repository content and generates suggested topics that repository admins can accept or reject. プライベートリポジトリの内容は分析されず、Topics が推奨されることはありません。{% endif %}
-パブリックリポジトリもプライベートリポジトリも Topics を持つことができますが、Topics の検索結果で見えるプライベートリポジトリはアクセス権を持っているものだけです。
+{% if currentVersion == "github-ae@latest" %}Internal {% else %}Public, internal, {% endif %}and private repositories can have topics, although you will only see private repositories that you have access to in topic search results.
特定のトピックに関連付けられているリポジトリを検索できます。 詳しい情報については[リポジトリの検索](/articles/searching-for-repositories#search-by-topic)を参照してください。 また、{% data variables.product.product_name %} 上でトピックのリストを検索することもできます。 詳細は「[トピックを検索する](/articles/searching-topics)」を参照してください。
diff --git a/translations/ja-JP/content/github/administering-a-repository/customizing-your-repositorys-social-media-preview.md b/translations/ja-JP/content/github/administering-a-repository/customizing-your-repositorys-social-media-preview.md
index a503e437ef73..ea52ae7947ed 100644
--- a/translations/ja-JP/content/github/administering-a-repository/customizing-your-repositorys-social-media-preview.md
+++ b/translations/ja-JP/content/github/administering-a-repository/customizing-your-repositorys-social-media-preview.md
@@ -11,7 +11,7 @@ versions:
画像を追加するまでは、リポジトリへのリンクは、リポジトリの基本的な情報とオーナーのアバターを表示します。 リポジトリに画像を追加すると、さまざまなソーシャルプラットフォーム上で、あなたのプロジェクトが見つかりやすくなります。
-画像はプライベートリポジトリにアップロードすることもできますが、共有できるのはパブリックリポジトリにアップロードしたもののみです。
+{% if currentVersion != "github-ae@latest" %}You can upload an image to a private repository, but your image can only be shared from a public repository.{% endif %}
{% tip %}
ヒント: 画像は、1 MB 未満の PNG、JPG または GIF である必要があります。 最高の画質を得るため、画像は 640 × 320 ピクセルに収めるようおすすめします。
diff --git a/translations/ja-JP/content/github/administering-a-repository/deleting-a-repository.md b/translations/ja-JP/content/github/administering-a-repository/deleting-a-repository.md
index be69237a1d54..96a89634c7de 100644
--- a/translations/ja-JP/content/github/administering-a-repository/deleting-a-repository.md
+++ b/translations/ja-JP/content/github/administering-a-repository/deleting-a-repository.md
@@ -13,17 +13,16 @@ versions:
{% data reusables.organizations.owners-and-admins-can %}Organization のリポジトリを削除できます。 [**Allow members to delete or transfer repositories for this organization**] が無効化されていると、Organization のオーナーだけが Organization のリポジトリを削除できます。 {% data reusables.organizations.new-repo-permissions-more-info %}
-{% if currentVersion == "free-pro-team@latest" %}
+{% if currentVersion != "github-ae@latest" %}Deleting a public repository will not delete any forks of the repository.{% endif %}
+
{% warning %}
-**警告**: リポジトリを削除すると、リリース添付ファイルとチーム権限が**完全に**削除されます。 このアクションは取り消すことが**できません**。
+**Warnings**:
-{% endwarning %}
-{% endif %}
+- Deleting a repository will **permanently** delete release attachments and team permissions. このアクションは取り消すことが**できません**。
+- Deleting a private {% if currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" %}or internal {% endif %}repository will delete all forks of the repository.
-次の点もご注意ください:
-- プライベートリポジトリを削除すると、そのフォークもすべて削除されます。
-- パブリックリポジトリを削除しても、そのフォークは削除されません。
+{% endwarning %}
{% if currentVersion == "free-pro-team@latest" %}
削除したリポジトリは、90 日以内であれば復元できます。 詳しい情報については、「[削除されたリポジトリを復元する](/articles/restoring-a-deleted-repository)」を参照してください。
diff --git a/translations/ja-JP/content/github/administering-a-repository/enabling-anonymous-git-read-access-for-a-repository.md b/translations/ja-JP/content/github/administering-a-repository/enabling-anonymous-git-read-access-for-a-repository.md
index 9dcf90757845..01d25e39e2f5 100644
--- a/translations/ja-JP/content/github/administering-a-repository/enabling-anonymous-git-read-access-for-a-repository.md
+++ b/translations/ja-JP/content/github/administering-a-repository/enabling-anonymous-git-read-access-for-a-repository.md
@@ -5,7 +5,6 @@ redirect_from:
- /articles/enabling-anonymous-git-read-access-for-a-repository
versions:
enterprise-server: '*'
- github-ae: '*'
---
次の場合に、リポジトリの管理者は、特定のリポジトリに対する匿名 Git 読み取りアクセスの設定を変更できます。
diff --git a/translations/ja-JP/content/github/administering-a-repository/managing-repository-settings.md b/translations/ja-JP/content/github/administering-a-repository/managing-repository-settings.md
index 650f1047e8b1..98776d347efc 100644
--- a/translations/ja-JP/content/github/administering-a-repository/managing-repository-settings.md
+++ b/translations/ja-JP/content/github/administering-a-repository/managing-repository-settings.md
@@ -1,6 +1,6 @@
---
title: リポジトリ設定を管理する
-intro: 'リポジトリの管理者と Organization のオーナーは、リポジトリの名前や所有権、リポジトリの可視性がパブリックかプライベートかなど、いくつかの設定を変更できます。 リポジトリを削除することもできます。'
+intro: 'Repository administrators and organization owners can change settings for a repository, like the name, ownership, and visibility, or delete the repository.'
mapTopic: true
redirect_from:
- /articles/managing-repository-settings
diff --git a/translations/ja-JP/content/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository.md b/translations/ja-JP/content/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository.md
index b433c5b5f690..743adbd6009d 100644
--- a/translations/ja-JP/content/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository.md
+++ b/translations/ja-JP/content/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository.md
@@ -22,28 +22,28 @@ versions:
{% data reusables.repositories.navigate-to-security-and-analysis %}
4. [Configure security and analysis features] で、機能の右側にある [**Disable**] または [**Enable**] をクリックします。 ![[Configure security and analysis] 機能の [Enable] または [Disable] ボタン](/assets/images/help/repository/security-and-analysis-disable-or-enable.png)
-### {% data variables.product.prodname_dependabot_alerts %} へのアクセスを許可する
+### セキュリティアラートへのアクセスを許可する
-Organization のリポジトリで {% data variables.product.prodname_dependabot_alerts %} を有効にすると、Organization のオーナーとリポジトリ管理者はデフォルトでアラートを表示できます。 追加の Team やユーザに、リポジトリのアラートへのアクセスを付与することができます。
+After you enable {% data variables.product.prodname_dependabot %} or {% data variables.product.prodname_secret_scanning %} alerts for a repository in an organization, organization owners and repository administrators can view the alerts by default. 追加の Team やユーザに、リポジトリのアラートへのアクセスを付与することができます。
{% note %}
-Organization owners and repository administrators can only grant access to view {% data variables.product.prodname_dependabot_alerts %} to people or teams who have write access to the repo.
+Organization owners and repository administrators can only grant access to view security alerts, such as {% data variables.product.prodname_dependabot %} and {% data variables.product.prodname_secret_scanning %} alerts, to people or teams who have write access to the repo.
{% endnote %}
{% data reusables.repositories.navigate-to-repo %}
{% data reusables.repositories.sidebar-settings %}
{% data reusables.repositories.navigate-to-security-and-analysis %}
-4. [Dependabot alerts] の検索フィールドで、検索するユーザまたは Team 名の入力を開始し、リストから一致する名前をクリックします。 
-5. [**Save changes**] をクリックします。 ![Dependabot アラート設定を変更するための [Save changes] ボタン](/assets/images/help/repository/security-and-analysis-security-alerts-save-changes.png)
+4. Under "Access to alerts", in the search field, start typing the name of the person or team you'd like to find, then click a name in the list of matches. 
+5. [**Save changes**] をクリックします。 
-### {% data variables.product.prodname_dependabot_alerts %} へのアクセスを削除する
+### セキュリティアラートへのアクセスを削除する
{% data reusables.repositories.navigate-to-repo %}
{% data reusables.repositories.sidebar-settings %}
{% data reusables.repositories.navigate-to-security-and-analysis %}
-4. [Dependabot alerts] で、アクセスを削除するユーザまたは Team の右側にある {% octicon "x" aria-label="X symbol" %} をクリックします。 ![リポジトリの Dependabot アラートへのユーザのアクセスを削除する [x] ボタン](/assets/images/help/repository/security-and-analysis-security-alerts-username-x.png)
+4. Under "Access to alerts", to the right of the person or team whose access you'd like to remove, click {% octicon "x" aria-label="X symbol" %}. 
### 参考リンク
diff --git a/translations/ja-JP/content/github/administering-a-repository/setting-repository-visibility.md b/translations/ja-JP/content/github/administering-a-repository/setting-repository-visibility.md
index ce0b2840cff1..3dcb634f52b4 100644
--- a/translations/ja-JP/content/github/administering-a-repository/setting-repository-visibility.md
+++ b/translations/ja-JP/content/github/administering-a-repository/setting-repository-visibility.md
@@ -19,17 +19,36 @@ Organization のオーナーは、リポジトリの可視性を変更する機
リポジトリの可視性を変更する前に、次の注意点を確認することをお勧めします。
#### リポジトリをプライベートにする
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+* {% data variables.product.product_name %} はパブリックリポジトリのパブリックフォークを切り離し、新しいネットワークに追加します。 Public forks are not made private.{% endif %}
+* If you change a repository's visibility from internal to private, {% data variables.product.prodname_dotcom %} will remove forks that belong to any user without access to the newly private repository. {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}The visibility of any forks will also change to private.{% elsif currentVersion == "github-ae@latest" %}If the internal repository has any forks, the visibility of the forks is already private.{% endif %} For more information, see "[What happens to forks when a repository is deleted or changes visibility?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility)"{% if currentVersion == "free-pro-team@latest" %}
+* If you're using {% data variables.product.prodname_free_user %} for user accounts or organizations, some features won't be available in the repository after you change the visibility to private. {% data reusables.gated-features.more-info %}{% endif %}
+* Any published {% data variables.product.prodname_pages %} site will be automatically unpublished.{% if currentVersion == "free-pro-team@latest" %} If you added a custom domain to the {% data variables.product.prodname_pages %} site, you should remove or update your DNS records before making the repository private, to avoid the risk of a domain takeover. For more information, see "[Managing a custom domain for your {% data variables.product.prodname_pages %} site](/articles/managing-a-custom-domain-for-your-github-pages-site)."{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+* 今後、{% data variables.product.prodname_dotcom %} は {% data variables.product.prodname_archive %} にリポジトリを含まなくなります。 For more information, see "[About archiving content and data on {% data variables.product.prodname_dotcom %}](/github/creating-cloning-and-archiving-repositories/about-archiving-content-and-data-on-github#about-the-github-archive-program)."{% endif %}{% if enterpriseServerVersions contains currentVersion %}
+* Anonymous Git read access is no longer available. For more information, see "[Enabling anonymous Git read access for a repository](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)."{% endif %}
- * {% data variables.product.prodname_dotcom %} はパブリックリポジトリのパブリックフォークを切り離し、新しいネットワークに追加します。 パブリックフォークはプライベートにはなりません。 {% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %}If you change a repository's visibility from internal to private, {% data variables.product.prodname_dotcom %} will remove forks that belong to any user without access to the newly private repository.{% endif %} For more information, see "[What happens to forks when a repository is deleted or changes visibility?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility#changing-a-public-repository-to-a-private-repository)"
- {% if currentVersion == "free-pro-team@latest" %}* If you're using {% data variables.product.prodname_free_user %} for user accounts or organizations, some features won't be available in the repository after you change the visibility to private. {% data reusables.gated-features.more-info %}
- * すべての公開済みの {% data variables.product.prodname_pages %} サイトは自動的に取り下げられます。 {% data variables.product.prodname_pages %} サイトにカスタムドメインを追加した場合、ドメインの乗っ取りリスクを回避するために、リポジトリをプライベートに設定する前に DNS レコードを削除または更新してください。 詳しい情報については、「[{% data variables.product.prodname_pages %} サイト用のカスタムドメインを管理する](/articles/managing-a-custom-domain-for-your-github-pages-site)」を参照してください。
- * 今後、{% data variables.product.prodname_dotcom %} は {% data variables.product.prodname_archive %} にリポジトリを含まなくなります。 詳しい情報については、「[{% data variables.product.prodname_dotcom %} のコンテンツとデータのアーカイブについて](/github/creating-cloning-and-archiving-repositories/about-archiving-content-and-data-on-github#about-the-github-archive-program)」を参照してください。{% endif %}
- {% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}* Anonymous Git read access is no longer available. 詳しい情報については、「[リポジトリで匿名 Git 読み取りアクセスを有効化する](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)」を参照してください。{% endif %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %}
+
+#### リポジトリをインターナルにする
+
+{% note %}
+
+**Note:** {% data reusables.gated-features.internal-repos %}
+
+{% endnote %}
+
+* Any forks of the repository will remain in the repository network, and {% data variables.product.product_name %} maintains the relationship between the root repository and the fork. For more information, see "[What happens to forks when a repository is deleted or changes visibility?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility)"
+
+{% endif %}
+
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
#### リポジトリをパブリックにする
- * {% data variables.product.prodname_dotcom %} はプライベートフォークを切り離し、スタンドアロンのプライベートリポジトリに変換します。 詳細は「[リポジトリが削除されたり可視性が変更されたりするとフォークはどうなりますか?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility#changing-a-private-repository-to-a-public-repository)」を参照してください。
- * If you're converting your private repository to a public repository as part of a move toward creating an open source project, see the [Open Source Guides](http://opensource.guide) for helpful tips and guidelines.{% if currentVersion == "free-pro-team@latest" %} You can also take a free course on managing an open source project with [{% data variables.product.prodname_learning %}]({% data variables.product.prodname_learning_link %}). リポジトリがパブリックになったら、コントリビューターをサポートするための最適な手法にプロジェクトが合致しているかどうかを確認するため、リポジトリのコミュニティプロフィールを表示できます。 詳細は「[コミュニティプロフィールを見る](/articles/viewing-your-community-profile)」を参照してください。{% endif %}
+* {% data variables.product.product_name %} will detach private forks and turn them into a standalone private repository. For more information, see "[What happens to forks when a repository is deleted or changes visibility?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility#changing-a-private-repository-to-a-public-repository)"{% if currentVersion == "free-pro-team@latest" %}
+* If you're converting your private repository to a public repository as part of a move toward creating an open source project, see the [Open Source Guides](http://opensource.guide) for helpful tips and guidelines. You can also take a free course on managing an open source project with [{% data variables.product.prodname_learning %}]({% data variables.product.prodname_learning_link %}). リポジトリがパブリックになったら、コントリビューターをサポートするための最適な手法にプロジェクトが合致しているかどうかを確認するため、リポジトリのコミュニティプロフィールを表示できます。 詳細は「[コミュニティプロフィールを見る](/articles/viewing-your-community-profile)」を参照してください。{% endif %}
+
+{% endif %}
{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
diff --git a/translations/ja-JP/content/github/authenticating-to-github/about-anonymized-image-urls.md b/translations/ja-JP/content/github/authenticating-to-github/about-anonymized-image-urls.md
index 529ccfd62ed2..d41e16a2bd7e 100644
--- a/translations/ja-JP/content/github/authenticating-to-github/about-anonymized-image-urls.md
+++ b/translations/ja-JP/content/github/authenticating-to-github/about-anonymized-image-urls.md
@@ -8,7 +8,7 @@ versions:
free-pro-team: '*'
---
-画像をホストするために、{% data variables.product.product_name %}は[オープンソースプロジェクトの Camo](https://github.com/atmos/camo) を使用します。 `https://camo.githubusercontent.com/` で始まる各画像に対して、Camo は匿名 URL プロキシを生成し、ブラウザの詳細や関連情報が他のユーザから見えないようにします。
+画像をホストするために、{% data variables.product.product_name %}は[オープンソースプロジェクトの Camo](https://github.com/atmos/camo) を使用します。 Camo generates an anonymous URL proxy for each image which hides your browser details and related information from other users. The URL starts `https://.githubusercontent.com/`, with different subdomains depending on how you uploaded the image.
匿名化した画像URLを受け取った人は、直接であれ間接であれ、その画像を見ることができます。 機密の画像をプライベートにしておきたい場合は、Camoを使う代わりにそれらを認証が必要なプライベートなネットワークあるいはサーバーから提供するようにしてください。
diff --git a/translations/ja-JP/content/github/authenticating-to-github/adding-a-new-ssh-key-to-your-github-account.md b/translations/ja-JP/content/github/authenticating-to-github/adding-a-new-ssh-key-to-your-github-account.md
index 68299bc87ba0..ac363d56f70b 100644
--- a/translations/ja-JP/content/github/authenticating-to-github/adding-a-new-ssh-key-to-your-github-account.md
+++ b/translations/ja-JP/content/github/authenticating-to-github/adding-a-new-ssh-key-to-your-github-account.md
@@ -24,8 +24,8 @@ versions:
使用する SSH キーの名前がサンプルのコードとは違っている場合は、現在の設定に合わせてファイル名を修正してください。 キーをコピーする際には、改行や空白を追加しないでください。
```shell
- $ pbcopy < ~/.ssh/id_rsa.pub
- # id_rsa.pub ファイルの内容をクリップボードにコピーする
+ $ pbcopy < ~/.ssh/id_ed25519.pub
+ # Copies the contents of the id_ed25519.pub file to your clipboard
```
{% tip %}
@@ -51,8 +51,8 @@ versions:
使用する SSH キーの名前がサンプルのコードとは違っている場合は、現在の設定に合わせてファイル名を修正してください。 キーをコピーする際には、改行や空白を追加しないでください。
```shell
- $ clip < ~/.ssh/id_rsa.pub
- # id_rsa.pub ファルの内容をクリップボードにコピーする
+ $ clip < ~/.ssh/id_ed25519.pub
+ # Copies the contents of the id_ed25519.pub file to your clipboard
```
{% tip %}
@@ -81,8 +81,8 @@ versions:
$ sudo apt-get install xclip
# xclip をダウンロードしてインストールします。 If you don't have `apt-get`, you might need to use another installer (like `yum`)
- $ xclip -selection clipboard < ~/.ssh/id_rsa.pub
- # Copies the contents of the id_rsa.pub file to your clipboard
+ $ xclip -selection clipboard < ~/.ssh/id_ed25519.pub
+ # Copies the contents of the id_ed25519.pub file to your clipboard
```
{% tip %}
diff --git a/translations/ja-JP/content/github/authenticating-to-github/generating-a-new-ssh-key-and-adding-it-to-the-ssh-agent.md b/translations/ja-JP/content/github/authenticating-to-github/generating-a-new-ssh-key-and-adding-it-to-the-ssh-agent.md
index 6c47b9b822c3..cfa15cc3e110 100644
--- a/translations/ja-JP/content/github/authenticating-to-github/generating-a-new-ssh-key-and-adding-it-to-the-ssh-agent.md
+++ b/translations/ja-JP/content/github/authenticating-to-github/generating-a-new-ssh-key-and-adding-it-to-the-ssh-agent.md
@@ -98,13 +98,19 @@ SSH キーを使用するたびにパスフレーズを再入力したくない
IdentityFile ~/.ssh/id_ed25519
```
+ {% note %}
+
+ **Note:** If you chose not to add a passphrase to your key, you should omit the `UseKeychain` line.
+
+ {% endnote %}
+
3. SSH 秘密鍵を ssh-agent に追加して、パスフレーズをキーチェーンに保存します。 {% data reusables.ssh.add-ssh-key-to-ssh-agent %}
```shell
$ ssh-add -K ~/.ssh/id_ed25519
```
{% note %}
- **メモ: ** `-K` オプションは、`ssh-add` の Apple の標準バージョン内にあり、ssh-agent に SSH キーを追加する際にキーチェーンにパスフレーズを保存します。
+ **メモ: ** `-K` オプションは、`ssh-add` の Apple の標準バージョン内にあり、ssh-agent に SSH キーを追加する際にキーチェーンにパスフレーズを保存します。 If you chose not to add a passphrase to your key, run the command without the `-K` option.
Apple の標準バージョンをインストールしていない場合は、エラーが発生する場合があります。 このエラーの解決方法についての詳細は、「[エラー: ssh-add: illegal option -- K](/articles/error-ssh-add-illegal-option-k)」を参照してください。
diff --git a/translations/ja-JP/content/github/authenticating-to-github/reviewing-your-security-log.md b/translations/ja-JP/content/github/authenticating-to-github/reviewing-your-security-log.md
index 5653f5e5e11d..cc63b95edbb3 100644
--- a/translations/ja-JP/content/github/authenticating-to-github/reviewing-your-security-log.md
+++ b/translations/ja-JP/content/github/authenticating-to-github/reviewing-your-security-log.md
@@ -1,6 +1,7 @@
---
title: セキュリティログをレビューする
intro: ユーザアカウントのセキュリティログをレビューして、自分が実行したアクションと、他のユーザが実行したアクションについて詳しく知ることができます。
+miniTocMaxHeadingLevel: 4
redirect_from:
- /articles/reviewing-your-security-log
versions:
@@ -30,216 +31,222 @@ The security log lists all actions performed within the last 90 days{% if curren
#### 実行されたアクションに基づく検索
{% else %}
### セキュリティログでのイベントを理解する
+{% endif %}
+
+The events listed in your security log are triggered by your actions. Actions are grouped into the following categories:
+
+| カテゴリ名 | 説明 |
+| -------------------------------------------------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |{% if currentVersion == "free-pro-team@latest" %}
+| [`account_recovery_token`](#account_recovery_token-category-actions) | | カテゴリ名 | 説明 [リカバリトークンの追加](/articles/configuring-two-factor-authentication-recovery-methods)に関連するすべての活動が対象です。 |
+| [`支払い`](#billing-category-actions) | 自分の支払い情報に関連するすべての活動が対象です。 |
+| [`marketplace_agreement_signature`](#marketplace_agreement_signature-category-actions) | {% data variables.product.prodname_marketplace %} Developer Agreement の署名に関連するすべての活動が対象です。 |
+| [`marketplace_listing`](#marketplace_listing-category-actions) | {% data variables.product.prodname_marketplace %} に一覧表示しているアプリに関連するすべての活動が対象です。{% endif %}
+| [`oauth_access`](#oauth_access-category-actions) | Contains all activities related to [{% data variables.product.prodname_oauth_app %}s](/articles/authorizing-oauth-apps) you've connected with.{% if currentVersion == "free-pro-team@latest" %}
+| [`payment_method`](#payment_method-category-actions) | {% data variables.product.prodname_dotcom %} プランに対する支払いに関連するすべての活動が対象です。{% endif %}
+| [`profile_picture`](#profile_picture-category-actions) | 自分のプロファイル写真に関連するすべての活動が対象です。 |
+| [`project`](#project-category-actions) | プロジェクト ボードに関連するすべての活動が対象です。 |
+| [`public_key`](#public_key-category-actions) | [公開 SSH キー](/articles/adding-a-new-ssh-key-to-your-github-account)に関連するすべての活動が対象です。 |
+| [`repo`](#repo-category-actions) | Contains all activities related to the repositories you own.{% if currentVersion == "free-pro-team@latest" %}
+| [`sponsors`](#sponsors-category-actions) | Contains all events related to {% data variables.product.prodname_sponsors %} and sponsor buttons (see "[About {% data variables.product.prodname_sponsors %}](/articles/about-github-sponsors)" and "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)"){% endif %}{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
+| [`Team`](#team-category-actions) | Contains all activities related to teams you are a part of.{% endif %}{% if currentVersion != "github-ae@latest" %}
+| [`two_factor_authentication`](#two_factor_authentication-category-actions) | Contains all activities related to [two-factor authentication](/articles/securing-your-account-with-two-factor-authentication-2fa).{% endif %}
+| [`ユーザ`](#user-category-actions) | アカウントに関連するすべての活動が対象です。 |
+
+{% if currentVersion == "free-pro-team@latest" %}
+
+### セキュリティログをエクスポートする
+
+{% data reusables.audit_log.export-log %}
+{% data reusables.audit_log.exported-log-keys-and-values %}
-セキュリティログに一覧表示されるアクションは以下のカテゴリに分類されます。 |{% endif %}
-| カテゴリー名 | 説明 |
-| ------------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |{% if currentVersion == "free-pro-team@latest" %}
-| `account_recovery_token` | [リカバリトークンの追加](/articles/configuring-two-factor-authentication-recovery-methods)に関連するすべての活動が対象です。 |
-| `支払い` | 自分の支払い情報に関連するすべての活動が対象です。 |
-| `marketplace_agreement_signature` | {% data variables.product.prodname_marketplace %} Developer Agreement の署名に関連するすべての活動が対象です。 |
-| `marketplace_listing` | {% data variables.product.prodname_marketplace %} に一覧表示しているアプリに関連するすべての活動が対象です。{% endif %}
-| `oauth_access` | Contains all activities related to [{% data variables.product.prodname_oauth_app %}s](/articles/authorizing-oauth-apps) you've connected with.{% if currentVersion == "free-pro-team@latest" %}
-| `payment_method` | {% data variables.product.prodname_dotcom %} プランに対する支払いに関連するすべての活動が対象です。{% endif %}
-| `profile_picture` | 自分のプロファイル写真に関連するすべての活動が対象です。 |
-| `project` | プロジェクト ボードに関連するすべての活動が対象です。 |
-| `public_key` | [公開 SSH キー](/articles/adding-a-new-ssh-key-to-your-github-account)に関連するすべての活動が対象です。 |
-| `repo` | Contains all activities related to the repositories you own.{% if currentVersion == "free-pro-team@latest" %}
-| `sponsors` | Contains all events related to {% data variables.product.prodname_sponsors %} and sponsor buttons (see "[About {% data variables.product.prodname_sponsors %}](/articles/about-github-sponsors)" and "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)"){% endif %}{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-| `Team` | Contains all activities related to teams you are a part of.{% endif %}{% if currentVersion != "github-ae@latest" %}
-| `two_factor_authentication` | Contains all activities related to [two-factor authentication](/articles/securing-your-account-with-two-factor-authentication-2fa).{% endif %}
-| `ユーザ` | アカウントに関連するすべての活動が対象です。 |
-
-これらのカテゴリ内のイベントの説明は以下のとおりです。
+{% endif %}
+
+### Security log actions
+
+An overview of some of the most common actions that are recorded as events in the security log.
{% if currentVersion == "free-pro-team@latest" %}
-#### `account_recovery_token` カテゴリ
+#### `account_recovery_token` category actions
-| アクション | 説明 |
-| ------------- | ----------------------------------------------------------------------------------------------------------- |
-| confirm | 正常に[リカバリプロバイダ付きの新たなトークンを保存する](/articles/configuring-two-factor-authentication-recovery-methods)ときにトリガーされます。 |
-| recover | 正常に[アカウント リカバリ トークンを引き換える](/articles/recovering-your-account-if-you-lose-your-2fa-credentials)ときにトリガーされます。 |
-| recover_error | トークンが使用されているにもかかわらず {% data variables.product.prodname_dotcom %} がそれを有効にできないときにトリガーされます。 |
+| アクション | 説明 |
+| --------------- | ----------------------------------------------------------------------------------------------------------- |
+| `confirm` | 正常に[リカバリプロバイダ付きの新たなトークンを保存する](/articles/configuring-two-factor-authentication-recovery-methods)ときにトリガーされます。 |
+| `recover` | 正常に[アカウント リカバリ トークンを引き換える](/articles/recovering-your-account-if-you-lose-your-2fa-credentials)ときにトリガーされます。 |
+| `recover_error` | トークンが使用されているにもかかわらず {% data variables.product.prodname_dotcom %} がそれを有効にできないときにトリガーされます。 |
-#### `billing` カテゴリ
+#### `billing` category actions
| アクション | 説明 |
| --------------------- | -------------------------------------------------------------------------------------------------------------------- |
-| change_billing_type | {% data variables.product.prodname_dotcom %} の[支払い方法を変更する](/articles/adding-or-editing-a-payment-method)ときにトリガーされます。 |
-| change_email | [自分のメール アドレスを変更する](/articles/changing-your-primary-email-address)ときにトリガーされます。 |
+| `change_billing_type` | {% data variables.product.prodname_dotcom %} の[支払い方法を変更する](/articles/adding-or-editing-a-payment-method)ときにトリガーされます。 |
+| `change_email` | [自分のメール アドレスを変更する](/articles/changing-your-primary-email-address)ときにトリガーされます。 |
-#### `marketplace_agreement_signature` カテゴリ
+#### `marketplace_agreement_signature` category actions
-| アクション | 説明 |
-| ------ | --------------------------------------------------------------------------------------- |
-| create | {% data variables.product.prodname_marketplace %} Developer Agreement に署名するときにトリガーされます。 |
+| アクション | 説明 |
+| -------- | --------------------------------------------------------------------------------------- |
+| `create` | {% data variables.product.prodname_marketplace %} Developer Agreement に署名するときにトリガーされます。 |
-#### `marketplace_listing` カテゴリ
+#### `marketplace_listing` category actions
-| アクション | 説明 |
-| ------- | ----------------------------------------------------------------------------------- |
-| 承認 | 一覧表を {% data variables.product.prodname_marketplace %}に掲載することが承認されるときにトリガーされます。 |
-| create | {% data variables.product.prodname_marketplace %} で自分のアプリケーションの一覧表を作成するときにトリガーされます。 |
-| delist | 一覧表が {% data variables.product.prodname_marketplace %} から削除されるときにトリガーされます。 |
-| redraft | 一覧表がドラフト状態に戻されるときにトリガーされます。 |
-| reject | 一覧表が {% data variables.product.prodname_marketplace %} に掲載することを認められないときにトリガーされます。 |
+| アクション | 説明 |
+| --------- | ----------------------------------------------------------------------------------- |
+| `承認` | 一覧表を {% data variables.product.prodname_marketplace %}に掲載することが承認されるときにトリガーされます。 |
+| `create` | {% data variables.product.prodname_marketplace %} で自分のアプリケーションの一覧表を作成するときにトリガーされます。 |
+| `delist` | 一覧表が {% data variables.product.prodname_marketplace %} から削除されるときにトリガーされます。 |
+| `redraft` | 一覧表がドラフト状態に戻されるときにトリガーされます。 |
+| `reject` | 一覧表が {% data variables.product.prodname_marketplace %} に掲載することを認められないときにトリガーされます。 |
{% endif %}
-#### `oauth_access` カテゴリ
+#### `oauth_access` category actions
-| アクション | 説明 |
-| ------- | ---------------------------------------------------------------------------------------------------------------------------------------- |
-| create | [{% data variables.product.prodname_oauth_app %} へのアクセスを許可する](/articles/authorizing-oauth-apps)ときにトリガーされます。 |
-| destroy | [自分のアカウントへの {% data variables.product.prodname_oauth_app %} のアクセス権を取り消す](/articles/reviewing-your-authorized-integrations)ときにトリガーされます。 |
+| アクション | 説明 |
+| --------- | ---------------------------------------------------------------------------------------------------------------------------------------- |
+| `create` | [{% data variables.product.prodname_oauth_app %} へのアクセスを許可する](/articles/authorizing-oauth-apps)ときにトリガーされます。 |
+| `destroy` | [自分のアカウントへの {% data variables.product.prodname_oauth_app %} のアクセス権を取り消す](/articles/reviewing-your-authorized-integrations)ときにトリガーされます。 |
{% if currentVersion == "free-pro-team@latest" %}
-#### `payment_method` カテゴリ
+#### `payment_method` category actions
-| アクション | 説明 |
-| ------ | -------------------------------------------------------------------- |
-| clear | ファイルでの[支払い方法](/articles/removing-a-payment-method)が削除されるときにトリガーされます。 |
-| create | 新しいクレジット カードや PayPal アカウントなど、新たな支払い方法が追加されるときにトリガーされます。 |
-| update | 既存の支払い方法が更新されるときにトリガーされます。 |
+| アクション | 説明 |
+| -------- | -------------------------------------------------------------------- |
+| `clear` | ファイルでの[支払い方法](/articles/removing-a-payment-method)が削除されるときにトリガーされます。 |
+| `create` | 新しいクレジット カードや PayPal アカウントなど、新たな支払い方法が追加されるときにトリガーされます。 |
+| `update` | 既存の支払い方法が更新されるときにトリガーされます。 |
{% endif %}
-#### `profile_picture` カテゴリ
+#### `profile_picture` category actions
-| アクション | 説明 |
-| ------ | ---------------------------------------------------------------------------- |
-| update | [自分のプロフィール写真を設定または更新する](/articles/setting-your-profile-picture/)ときにトリガーされます。 |
+| アクション | 説明 |
+| -------- | ---------------------------------------------------------------------------- |
+| `update` | [自分のプロフィール写真を設定または更新する](/articles/setting-your-profile-picture/)ときにトリガーされます。 |
-#### `project` カテゴリ
+#### `project` category actions
| アクション | 説明 |
| ------------------------ | ------------------------------------------------------------------------------------------------------------------------- |
+| `access` | プロジェクト ボードの可視性が変更されるときにトリガーされます。 |
| `create` | プロジェクト ボードが作成されるときにトリガーされます。 |
| `rename` | プロジェクトボードの名前が変更されるときにトリガーされます。 |
| `update` | プロジェクト ボードが更新されるときにトリガーされます。 |
| `delete` | プロジェクトボードが削除されるときにトリガーされます。 |
| `link` | リポジトリがプロジェクト ボードにリンクされるときにトリガーされます。 |
| `unlink` | リポジトリがプロジェクトボードからリンク解除されるときにトリガーされます。 |
-| `project.access` | プロジェクト ボードの可視性が変更されるときにトリガーされます。 |
| `update_user_permission` | Triggered when an outside collaborator is added to or removed from a project board or has their permission level changed. |
-#### `public_key` カテゴリ
+#### `public_key` category actions
-| アクション | 説明 |
-| ------ | ------------------------------------------------------------------------------------------------------------------------------------------ |
-| create | [新たな公開 SSH キーを自分の {% data variables.product.product_name %} アカウントに追加する](/articles/adding-a-new-ssh-key-to-your-github-account)ときにトリガーされます。 |
-| delete | [公開 SSH キーを自分の {% data variables.product.product_name %} アカウントから削除する](/articles/reviewing-your-ssh-keys)ときにトリガーされます。 |
+| アクション | 説明 |
+| -------- | ------------------------------------------------------------------------------------------------------------------------------------------ |
+| `create` | [新たな公開 SSH キーを自分の {% data variables.product.product_name %} アカウントに追加する](/articles/adding-a-new-ssh-key-to-your-github-account)ときにトリガーされます。 |
+| `delete` | [公開 SSH キーを自分の {% data variables.product.product_name %} アカウントから削除する](/articles/reviewing-your-ssh-keys)ときにトリガーされます。 |
-#### `repo` カテゴリ
+#### `repo` category actions
| アクション | 説明 |
| ------------------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| access | 自分が所有するリポジトリが["プライベート" から "パブリック" に切り替えられる](/articles/making-a-private-repository-public) (またはその逆) ときにトリガーされます。 |
-| add_member | Triggered when a {% data variables.product.product_name %} user is {% if currentVersion == "free-pro-team@latest" %}[invited to have collaboration access](/articles/inviting-collaborators-to-a-personal-repository){% else %}[given collaboration access](/articles/inviting-collaborators-to-a-personal-repository){% endif %} to a repository. |
-| add_topic | リポジトリのオーナーがリポジトリに[トピックを追加する](/articles/classifying-your-repository-with-topics)ときにトリガーされます。 |
-| archived | Triggered when a repository owner [archives a repository](/articles/about-archiving-repositories).{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-| config.disable_anonymous_git_access | 公開リポジトリで[匿名の Git 読み取りアクセスが無効になる](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)ときにトリガーされます。 |
-| config.enable_anonymous_git_access | 公開リポジトリで[匿名の Git 読み取りアクセスが有効になる](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)ときにトリガーされます。 |
-| config.lock_anonymous_git_access | リポジトリの[匿名の Git 読み取りアクセス設定がロックされる](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)ときにトリガーされます。 |
-| config.unlock_anonymous_git_access | リポジトリの[匿名の Git 読み取りアクセス設定がロック解除される](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)ときにトリガーされます。{% endif %}
-| create | [新たなリポジトリが作成される](/articles/creating-a-new-repository)ときにトリガーされます。 |
-| destroy | Triggered when [a repository is deleted](/articles/deleting-a-repository).{% if currentVersion == "free-pro-team@latest" %}
-| disable | Triggered when a repository is disabled (e.g., for [insufficient funds](/articles/unlocking-a-locked-account)).{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-| enable | リポジトリが再び有効になるときにトリガーされます。{% endif %}
-| remove_member | {% data variables.product.product_name %}ユーザが[リポジトリのコラボレーターではなくなる](/articles/removing-a-collaborator-from-a-personal-repository)ときにトリガーされます。 |
-| remove_topic | リポジトリのオーナーがリポジトリからトピックを削除するときにトリガーされます。 |
-| rename | [リポジトリの名前が変更される](/articles/renaming-a-repository)ときにトリガーされます。 |
-| 移譲 | [リポジトリが移譲される](/articles/how-to-transfer-a-repository)ときにトリガーされます。 |
-| transfer_start | リポジトリの移譲が行われようとしているときにトリガーされます。 |
-| unarchived | リポジトリのオーナーがリポジトリをアーカイブ解除するときにトリガーされます。 |
+| `access` | 自分が所有するリポジトリが["プライベート" から "パブリック" に切り替えられる](/articles/making-a-private-repository-public) (またはその逆) ときにトリガーされます。 |
+| `add_member` | Triggered when a {% data variables.product.product_name %} user is {% if currentVersion == "free-pro-team@latest" %}[invited to have collaboration access](/articles/inviting-collaborators-to-a-personal-repository){% else %}[given collaboration access](/articles/inviting-collaborators-to-a-personal-repository){% endif %} to a repository. |
+| `add_topic` | リポジトリのオーナーがリポジトリに[トピックを追加する](/articles/classifying-your-repository-with-topics)ときにトリガーされます。 |
+| `archived` | リポジトリのオーナーが[リポジトリをアーカイブする](/articles/about-archiving-repositories)ときにトリガーされます。{% if enterpriseServerVersions contains currentVersion %}
+| `config.disable_anonymous_git_access` | 公開リポジトリで[匿名の Git 読み取りアクセスが無効になる](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)ときにトリガーされます。 |
+| `config.enable_anonymous_git_access` | 公開リポジトリで[匿名の Git 読み取りアクセスが有効になる](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)ときにトリガーされます。 |
+| `config.lock_anonymous_git_access` | リポジトリの[匿名の Git 読み取りアクセス設定がロックされる](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)ときにトリガーされます。 |
+| `config.unlock_anonymous_git_access` | リポジトリの[匿名の Git 読み取りアクセス設定がロック解除される](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)ときにトリガーされます。{% endif %}
+| `create` | [新たなリポジトリが作成される](/articles/creating-a-new-repository)ときにトリガーされます。 |
+| `destroy` | Triggered when [a repository is deleted](/articles/deleting-a-repository).{% if currentVersion == "free-pro-team@latest" %}
+| `disable` | Triggered when a repository is disabled (e.g., for [insufficient funds](/articles/unlocking-a-locked-account)).{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| `enable` | リポジトリが再び有効になるときにトリガーされます。{% endif %}
+| `remove_member` | {% data variables.product.product_name %}ユーザが[リポジトリのコラボレーターではなくなる](/articles/removing-a-collaborator-from-a-personal-repository)ときにトリガーされます。 |
+| `remove_topic` | リポジトリのオーナーがリポジトリからトピックを削除するときにトリガーされます。 |
+| `rename` | [リポジトリの名前が変更される](/articles/renaming-a-repository)ときにトリガーされます。 |
+| `移譲` | [リポジトリが移譲される](/articles/how-to-transfer-a-repository)ときにトリガーされます。 |
+| `transfer_start` | リポジトリの移譲が行われようとしているときにトリガーされます。 |
+| `unarchived` | リポジトリのオーナーがリポジトリをアーカイブ解除するときにトリガーされます。 |
{% if currentVersion == "free-pro-team@latest" %}
-#### `sponsors` カテゴリ
-
-| アクション | 説明 |
-| ----------------------------------------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| repo_funding_link_button_toggle | リポジトリでスポンサーボタンの表示を有効化または無効化したときにトリガーされます (「[リポジトリにスポンサーボタンを表示する](/articles/displaying-a-sponsor-button-in-your-repository)」を参照) |
-| repo_funding_links_file_action | リポジトリで FUNDING ファイルを変更したときにトリガーされます (「[リポジトリにスポンサーボタンを表示する](/articles/displaying-a-sponsor-button-in-your-repository)」を参照) |
-| sponsor_sponsorship_cancel | スポンサーシップをキャンセルしたときにトリガーされます (「[スポンサーシップをダウングレードする](/articles/downgrading-a-sponsorship)」を参照) |
-| sponsor_sponsorship_create | 開発者をスポンサーするとトリガーされます (「[オープンソースコントリビューターに対するスポンサー](/github/supporting-the-open-source-community-with-github-sponsors/sponsoring-an-open-source-contributor#sponsoring-a-developer)」を参照) |
-| sponsor_sponsorship_preference_change | スポンサード開発者からメールで最新情報を受け取るかどうかを変更したときにトリガーされます (「[スポンサーシップを管理する](/articles/managing-your-sponsorship)」を参照) |
-| sponsor_sponsorship_tier_change | スポンサーシップをアップグレードまたはダウングレードしたときにトリガーされます (「[スポンサーシップをアップグレードする](/articles/upgrading-a-sponsorship)」および「[スポンサーシップをダウングレードする](/articles/downgrading-a-sponsorship)」を参照) |
-| sponsored_developer_approve | {% data variables.product.prodname_sponsors %}アカウントが承認されるとトリガーされます(「[ユーザアカウントに{% data variables.product.prodname_sponsors %}を設定する](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)」を参照) |
-| sponsored_developer_create | {% data variables.product.prodname_sponsors %}アカウントが作成されるとトリガーされます(「[ユーザアカウントに{% data variables.product.prodname_sponsors %}を設定する](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)」を参照) |
-| sponsored_developer_profile_update | スポンサード開発者のプロフィールを編集するとトリガーされます(「[{% data variables.product.prodname_sponsors %}のプロフィール詳細を編集する](/github/supporting-the-open-source-community-with-github-sponsors/editing-your-profile-details-for-github-sponsors)」を参照) |
-| sponsored_developer_request_approval | 承認のために{% data variables.product.prodname_sponsors %}のアプリケーションをサブミットするとトリガーされます(「[ユーザアカウントに{% data variables.product.prodname_sponsors %}を設定する](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)」を参照) |
-| sponsored_developer_tier_description_update | スポンサーシップ層の説明を変更したときにトリガーされます (「[スポンサーシップ層を変更する](/articles/changing-your-sponsorship-tiers)」を参照) |
-| sponsored_developer_update_newsletter_send | スポンサーにメールで最新情報を送信したときにトリガーされます (「[スポンサーに連絡する](/articles/contacting-your-sponsors)」を参照) |
-| waitlist_invite_sponsored_developer | 待ちリストから{% data variables.product.prodname_sponsors %}に参加するよう招待されたときにトリガーされます(「[ユーザアカウントに{% data variables.product.prodname_sponsors %}を設定する](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)」を参照) |
-| waitlist_join | スポンサード開発者になるために待ちリストに参加するとトリガーされます(「[ユーザアカウントに{% data variables.product.prodname_sponsors %}を設定する](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)」を参照) |
+#### `sponsors` category actions
+
+| アクション | 説明 |
+| --------------------------------------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `repo_funding_link_button_toggle` | リポジトリでスポンサーボタンの表示を有効化または無効化したときにトリガーされます (「[リポジトリにスポンサーボタンを表示する](/articles/displaying-a-sponsor-button-in-your-repository)」を参照) |
+| `repo_funding_links_file_action` | リポジトリで FUNDING ファイルを変更したときにトリガーされます (「[リポジトリにスポンサーボタンを表示する](/articles/displaying-a-sponsor-button-in-your-repository)」を参照) |
+| `sponsor_sponsorship_cancel` | スポンサーシップをキャンセルしたときにトリガーされます (「[スポンサーシップをダウングレードする](/articles/downgrading-a-sponsorship)」を参照) |
+| `sponsor_sponsorship_create` | 開発者をスポンサーするとトリガーされます (「[オープンソースコントリビューターに対するスポンサー](/github/supporting-the-open-source-community-with-github-sponsors/sponsoring-an-open-source-contributor#sponsoring-a-developer)」を参照) |
+| `sponsor_sponsorship_preference_change` | スポンサード開発者からメールで最新情報を受け取るかどうかを変更したときにトリガーされます (「[スポンサーシップを管理する](/articles/managing-your-sponsorship)」を参照) |
+| `sponsor_sponsorship_tier_change` | スポンサーシップをアップグレードまたはダウングレードしたときにトリガーされます (「[スポンサーシップをアップグレードする](/articles/upgrading-a-sponsorship)」および「[スポンサーシップをダウングレードする](/articles/downgrading-a-sponsorship)」を参照) |
+| `sponsored_developer_approve` | {% data variables.product.prodname_sponsors %}アカウントが承認されるとトリガーされます(「[ユーザアカウントに{% data variables.product.prodname_sponsors %}を設定する](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)」を参照) |
+| `sponsored_developer_create` | {% data variables.product.prodname_sponsors %}アカウントが作成されるとトリガーされます(「[ユーザアカウントに{% data variables.product.prodname_sponsors %}を設定する](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)」を参照) |
+| `sponsored_developer_profile_update` | スポンサード開発者のプロフィールを編集するとトリガーされます(「[{% data variables.product.prodname_sponsors %}のプロフィール詳細を編集する](/github/supporting-the-open-source-community-with-github-sponsors/editing-your-profile-details-for-github-sponsors)」を参照) |
+| `sponsored_developer_request_approval` | 承認のために{% data variables.product.prodname_sponsors %}のアプリケーションをサブミットするとトリガーされます(「[ユーザアカウントに{% data variables.product.prodname_sponsors %}を設定する](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)」を参照) |
+| `sponsored_developer_tier_description_update` | スポンサーシップ層の説明を変更したときにトリガーされます (「[スポンサーシップ層を変更する](/articles/changing-your-sponsorship-tiers)」を参照) |
+| `sponsored_developer_update_newsletter_send` | スポンサーにメールで最新情報を送信したときにトリガーされます (「[スポンサーに連絡する](/articles/contacting-your-sponsors)」を参照) |
+| `waitlist_invite_sponsored_developer` | 待ちリストから{% data variables.product.prodname_sponsors %}に参加するよう招待されたときにトリガーされます(「[ユーザアカウントに{% data variables.product.prodname_sponsors %}を設定する](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)」を参照) |
+| `waitlist_join` | スポンサード開発者になるために待ちリストに参加するとトリガーされます(「[ユーザアカウントに{% data variables.product.prodname_sponsors %}を設定する](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)」を参照) |
{% endif %}
{% if currentVersion == "free-pro-team@latest" %}
-#### `successor_invitation` カテゴリ
-
-| アクション | 説明 |
-| ------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| accept | 継承の招待を承諾するとトリガーされます(「[ユーザアカウントのリポジトリの所有権の継続性を管理する](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)」を参照) |
-| cancel | 継承の招待をキャンセルするとトリガーされます(「[ユーザアカウントのリポジトリの所有権の継続性を管理する](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)」を参照) |
-| create | 継承の招待を作成するとトリガーされます(「[ユーザアカウントのリポジトリの所有権の継続性を管理する](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)」を参照) |
-| decline | 継承の招待を拒否するとトリガーされます(「[ユーザアカウントのリポジトリの所有権の継続性を管理する](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)」を参照) |
-| revoke | 継承の招待を取り消すとトリガーされます(「[ユーザアカウントのリポジトリの所有権の継続性を管理する](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)」を参照) |
+#### `successor_invitation` category actions
+
+| アクション | 説明 |
+| --------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `accept` | 継承の招待を承諾するとトリガーされます(「[ユーザアカウントのリポジトリの所有権の継続性を管理する](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)」を参照) |
+| `cancel` | 継承の招待をキャンセルするとトリガーされます(「[ユーザアカウントのリポジトリの所有権の継続性を管理する](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)」を参照) |
+| `create` | 継承の招待を作成するとトリガーされます(「[ユーザアカウントのリポジトリの所有権の継続性を管理する](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)」を参照) |
+| `decline` | 継承の招待を拒否するとトリガーされます(「[ユーザアカウントのリポジトリの所有権の継続性を管理する](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)」を参照) |
+| `revoke` | 継承の招待を取り消すとトリガーされます(「[ユーザアカウントのリポジトリの所有権の継続性を管理する](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)」を参照) |
{% endif %}
{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-#### `team` カテゴリ
+#### `team` category actions
-| アクション | 説明 |
-| ----------------- | ------------------------------------------------------------------------------------------------------------- |
-| add_member | 自分が所属している Organization のメンバーが[自分を Team に追加する](/articles/adding-organization-members-to-a-team)ときにトリガーされます。 |
-| add_repository | 自分がメンバーである Team にリポジトリの管理が任せられるときにトリガーされます。 |
-| create | 自分が所属している Organization で新たな Team が作成されるときにトリガーされます。 |
-| destroy | 自分がメンバーである Team が Organization から削除されるときにトリガーされます。 |
-| remove_member | Organization のメンバーが自分がメンバーである [Team から削除される](/articles/removing-organization-members-from-a-team)ときにトリガーされます。 |
-| remove_repository | リポジトリが Team の管理下でなくなるときにトリガーされます。 |
+| アクション | 説明 |
+| ------------------- | ------------------------------------------------------------------------------------------------------------- |
+| `add_member` | 自分が所属している Organization のメンバーが[自分を Team に追加する](/articles/adding-organization-members-to-a-team)ときにトリガーされます。 |
+| `add_repository` | 自分がメンバーである Team にリポジトリの管理が任せられるときにトリガーされます。 |
+| `create` | 自分が所属している Organization で新たな Team が作成されるときにトリガーされます。 |
+| `destroy` | 自分がメンバーである Team が Organization から削除されるときにトリガーされます。 |
+| `remove_member` | Organization のメンバーが自分がメンバーである [Team から削除される](/articles/removing-organization-members-from-a-team)ときにトリガーされます。 |
+| `remove_repository` | リポジトリが Team の管理下でなくなるときにトリガーされます。 |
{% endif %}
{% if currentVersion != "github-ae@latest" %}
-#### `two_factor_authentication` カテゴリ
+#### `two_factor_authentication` category actions
-| アクション | 説明 |
-| -------- | ---------------------------------------------------------------------------------------------- |
-| enabled | [2 要素認証](/articles/securing-your-account-with-two-factor-authentication-2fa)が有効になるときにトリガーされます。 |
-| disabled | 2 要素認証が無効になるときにトリガーされます。 |
+| アクション | 説明 |
+| ---------- | ---------------------------------------------------------------------------------------------- |
+| `enabled` | [2 要素認証](/articles/securing-your-account-with-two-factor-authentication-2fa)が有効になるときにトリガーされます。 |
+| `disabled` | 2 要素認証が無効になるときにトリガーされます。 |
{% endif %}
-#### `user` カテゴリ
-
-| アクション | 説明 |
-| ---------------------------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| add_email | Triggered when you {% if currentVersion != "github-ae@latest" %}[add a new email address](/articles/changing-your-primary-email-address){% else %}add a new email address{% endif %}. |
-| create | 新たなユーザー アカウントを作成するときにトリガーされます。 |
-| remove_email | メール アドレスを削除するときにトリガーされます。 |
-| rename | Triggered when you rename your account.{% if currentVersion != "github-ae@latest" %}
-| change_password | 自分のパスワードを変更するときにトリガーされます。 |
-| forgot_password | Triggered when you ask for [a password reset](/articles/how-can-i-reset-my-password).{% endif %}
-| login | {% data variables.product.product_location %} にログインするときにトリガーされます |
-| failed_login | Triggered when you failed to log in successfully.{% if currentVersion != "github-ae@latest" %}
-| two_factor_requested | Triggered when {% data variables.product.product_name %} asks you for [your two-factor authentication code](/articles/accessing-github-using-two-factor-authentication).{% endif %}
-| show_private_contributions_count | [自分のプロフィールでプライベート コントリビューションを公開する](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)ときにトリガーされます。 |
-| hide_private_contributions_count | Triggered when you [hide private contributions on your profile](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile).{% if currentVersion == "free-pro-team@latest" %}
-| report_content | [Issue または Pull Request、あるいは Issue、Pull Request、または Commit でのコメントを報告する](/articles/reporting-abuse-or-spam)ときにトリガーされます。{% endif %}
-
-#### `user_status` カテゴリ
-
-| アクション | 説明 |
-| ------- | ------------------------------------------------------------------------------------------------------------------------- |
-| update | 自分のプロファイルでステータスを設定または変更するときにトリガーされます。 詳細は「[ステータスを設定する](/articles/personalizing-your-profile/#setting-a-status)」を参照してください。 |
-| destroy | 自分のプロファイルでステータスを消去するときにトリガーされます。 |
+#### `user` category actions
-{% if currentVersion == "free-pro-team@latest" %}
+| アクション | 説明 |
+| ---------------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `add_email` | Triggered when you {% if currentVersion != "github-ae@latest" %}[add a new email address](/articles/changing-your-primary-email-address){% else %}add a new email address{% endif %}. |
+| `create` | Triggered when you create a new user account.{% if currentVersion != "github-ae@latest" %}
+| `change_password` | 自分のパスワードを変更するときにトリガーされます。 |
+| `forgot_password` | Triggered when you ask for [a password reset](/articles/how-can-i-reset-my-password).{% endif %}
+| `hide_private_contributions_count` | [自分のプロファイルでプライベート コントリビューションを非表示にする](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)ときにトリガーされます。 |
+| `login` | {% data variables.product.product_location %} にログインするときにトリガーされます |
+| `failed_login` | 正常にログインできなかったときにトリガーされます |
+| `remove_email` | メール アドレスを削除するときにトリガーされます。 |
+| `rename` | Triggered when you rename your account.{% if currentVersion == "free-pro-team@latest" %}
+| `report_content` | [Issue または Pull Request、あるいは Issue、Pull Request、または Commit でのコメントを報告する](/articles/reporting-abuse-or-spam)ときにトリガーされます。{% endif %}
+| `show_private_contributions_count` | Triggered when you [publicize private contributions on your profile](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile).{% if currentVersion != "github-ae@latest" %}
+| `two_factor_requested` | Triggered when {% data variables.product.product_name %} asks you for [your two-factor authentication code](/articles/accessing-github-using-two-factor-authentication).{% endif %}
-### セキュリティログをエクスポートする
+#### `user_status` category actions
+
+| アクション | 説明 |
+| --------- | ------------------------------------------------------------------------------------------------------------------------- |
+| `update` | 自分のプロファイルでステータスを設定または変更するときにトリガーされます。 詳細は「[ステータスを設定する](/articles/personalizing-your-profile/#setting-a-status)」を参照してください。 |
+| `destroy` | 自分のプロファイルでステータスを消去するときにトリガーされます。 |
-{% data reusables.audit_log.export-log %}
-{% data reusables.audit_log.exported-log-keys-and-values %}
-{% endif %}
diff --git a/translations/ja-JP/content/github/building-a-strong-community/about-issue-and-pull-request-templates.md b/translations/ja-JP/content/github/building-a-strong-community/about-issue-and-pull-request-templates.md
index 74d19b419fad..565f26877a86 100644
--- a/translations/ja-JP/content/github/building-a-strong-community/about-issue-and-pull-request-templates.md
+++ b/translations/ja-JP/content/github/building-a-strong-community/about-issue-and-pull-request-templates.md
@@ -11,7 +11,11 @@ versions:
リポジトリでIssueやプルリクエストのテンプレートを作成すると、コントリビューターはそのテンプレートを使い、リポジトリのコントリビューションのガイドラインに沿ってIssuelをオープンしたり、プルリクエスト中の変更を提案したりできるようになります。 リポジトリへのコントリビューションのガイドラインの追加に関する詳しい情報については[リポジトリコントリビューターのためのガイドラインを定める](/articles/setting-guidelines-for-repository-contributors)を参照してください。
-You can create default issue and pull request templates for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. 詳しい情報については「[デフォルトのコミュニティ健全性ファイルを作成する](/github/building-a-strong-community/creating-a-default-community-health-file)」を参照してください。
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+You can create default issue and pull request templates for your organization or user account. 詳しい情報については「[デフォルトのコミュニティ健全性ファイルを作成する](/github/building-a-strong-community/creating-a-default-community-health-file)」を参照してください。
+
+{% endif %}
### Issueのテンプレート
diff --git a/translations/ja-JP/content/github/building-a-strong-community/about-wikis.md b/translations/ja-JP/content/github/building-a-strong-community/about-wikis.md
index 4f8718eb6208..37d1af974304 100644
--- a/translations/ja-JP/content/github/building-a-strong-community/about-wikis.md
+++ b/translations/ja-JP/content/github/building-a-strong-community/about-wikis.md
@@ -15,9 +15,9 @@ versions:
ウィキでは、{% data variables.product.product_name %} のあらゆる他の場所と同じようにコンテンツを書くことができます。 詳細は「[{% data variables.product.prodname_dotcom %} で書き、フォーマットしてみる](/articles/getting-started-with-writing-and-formatting-on-github)」を参照してください。 私たちは、さまざまなフォーマットを HTML に変更するのに[私たちのオープンソースマークアップライブラリ](https://github.com/github/markup)を使っているので、Markdown あるいはその他任意のサポートされているフォーマットで書くことができます。
-パブリックリポジトリ内のウィキはパブリックに利用でき、プライベートリポジトリではリポジトリにアクセスできる人々に限定されます。 詳細は「[リポジトリの可視性を設定する](/articles/setting-repository-visibility)」を参照してください。
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}If you create a wiki in a public repository, the wiki is available to {% if enterpriseServerVersions contains currentVersion %}anyone with access to {% data variables.product.product_location %}{% else %}the public{% endif %}. {% endif %}If you create a wiki in an internal or private repository, {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}people{% elsif currentVersion == "github-ae@latest" %}enterprise members{% endif %} with access to the repository can also access the wiki. 詳細は「[リポジトリの可視性を設定する](/articles/setting-repository-visibility)」を参照してください。
-ウィキは、{% data variables.product.product_name %} 上で直接編集することも、ウィキのファイルをローカルで編集することもできます。 デフォルトでは、リポジトリに書き込みアクセスを持っている人だけがウィキを変更できますが、パブリックリポジトリ内のウィキには {% data variables.product.product_name %} 上の誰もがコントリビューションできるようにすることができます。 詳細は「[ウィキへのアクセス権限を変更する](/articles/changing-access-permissions-for-wikis)」を参照してください。
+ウィキは、{% data variables.product.product_name %} 上で直接編集することも、ウィキのファイルをローカルで編集することもできます。 By default, only people with write access to your repository can make changes to wikis, although you can allow everyone on {% data variables.product.product_location %} to contribute to a wiki in {% if currentVersion == "github-ae@latest" %}an internal{% else %}a public{% endif %} repository. 詳細は「[ウィキへのアクセス権限を変更する](/articles/changing-access-permissions-for-wikis)」を参照してください。
### 参考リンク
diff --git a/translations/ja-JP/content/github/building-a-strong-community/adding-a-license-to-a-repository.md b/translations/ja-JP/content/github/building-a-strong-community/adding-a-license-to-a-repository.md
index 762d79dbb8c7..2e4912a9a38b 100644
--- a/translations/ja-JP/content/github/building-a-strong-community/adding-a-license-to-a-repository.md
+++ b/translations/ja-JP/content/github/building-a-strong-community/adding-a-license-to-a-repository.md
@@ -6,7 +6,6 @@ redirect_from:
versions:
free-pro-team: '*'
enterprise-server: '*'
- github-ae: '*'
---
リポジトリに見つけやすいライセンスを含めておくと、リポジトリにアクセスした人はリポジトリページの先頭ですぐに見つけることができます。 ライセンスファイル全体を読むには、ライセンスの名前をクリックします。
diff --git a/translations/ja-JP/content/github/building-a-strong-community/adding-support-resources-to-your-project.md b/translations/ja-JP/content/github/building-a-strong-community/adding-support-resources-to-your-project.md
index 7128fcf98877..6aa851d6d6fa 100644
--- a/translations/ja-JP/content/github/building-a-strong-community/adding-support-resources-to-your-project.md
+++ b/translations/ja-JP/content/github/building-a-strong-community/adding-support-resources-to-your-project.md
@@ -13,7 +13,11 @@ versions:

-You can create default support resources for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. 詳しい情報については「[デフォルトのコミュニティ健全性ファイルを作成する](/github/building-a-strong-community/creating-a-default-community-health-file)」を参照してください。
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+You can create default support resources for your organization or user account. 詳しい情報については「[デフォルトのコミュニティ健全性ファイルを作成する](/github/building-a-strong-community/creating-a-default-community-health-file)」を参照してください。
+
+{% endif %}
{% tip %}
diff --git a/translations/ja-JP/content/github/building-a-strong-community/configuring-issue-templates-for-your-repository.md b/translations/ja-JP/content/github/building-a-strong-community/configuring-issue-templates-for-your-repository.md
index ab0f225d02f7..a6039533d076 100644
--- a/translations/ja-JP/content/github/building-a-strong-community/configuring-issue-templates-for-your-repository.md
+++ b/translations/ja-JP/content/github/building-a-strong-community/configuring-issue-templates-for-your-repository.md
@@ -10,10 +10,16 @@ versions:
github-ae: '*'
---
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
{% data reusables.repositories.default-issue-templates %}
+{% endif %}
+
{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %}
+
### Issue テンプレートを作成する
+
{% endif %}
{% data reusables.repositories.navigate-to-repo %}
diff --git a/translations/ja-JP/content/github/building-a-strong-community/creating-a-default-community-health-file.md b/translations/ja-JP/content/github/building-a-strong-community/creating-a-default-community-health-file.md
index 10d095995676..6f77705ea3e4 100644
--- a/translations/ja-JP/content/github/building-a-strong-community/creating-a-default-community-health-file.md
+++ b/translations/ja-JP/content/github/building-a-strong-community/creating-a-default-community-health-file.md
@@ -7,7 +7,6 @@ redirect_from:
versions:
free-pro-team: '*'
enterprise-server: '*'
- github-ae: '*'
---
### デフォルトのコミュニティ健全性ファイルについて
diff --git a/translations/ja-JP/content/github/building-a-strong-community/creating-a-pull-request-template-for-your-repository.md b/translations/ja-JP/content/github/building-a-strong-community/creating-a-pull-request-template-for-your-repository.md
index 3395ee2e03ca..b6aa94a1f8ae 100644
--- a/translations/ja-JP/content/github/building-a-strong-community/creating-a-pull-request-template-for-your-repository.md
+++ b/translations/ja-JP/content/github/building-a-strong-community/creating-a-pull-request-template-for-your-repository.md
@@ -13,7 +13,11 @@ versions:
サポートしているどのフォルダにでも *PULL_REQUEST_TEMPLATE/* サブディレクトリを作成し、プルリクエストテンプレートを複数含めることができます。また、`template` クエリパラメータでプルリクエストの本文に使用するテンプレートを指定できます。 詳細は「[クエリパラメータによる Issue およびプルリクエストの自動化について](/articles/about-automation-for-issues-and-pull-requests-with-query-parameters)」を参照してください。
-You can create default pull request templates for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. 詳しい情報については「[デフォルトのコミュニティ健全性ファイルを作成する](/github/building-a-strong-community/creating-a-default-community-health-file)」を参照してください。
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+You can create default pull request templates for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. 詳しい情報については「[デフォルトのコミュニティ健全性ファイルを作成する](/github/building-a-strong-community/creating-a-default-community-health-file)」を参照してください。
+
+{% endif %}
### プルリクエストテンプレートの追加
diff --git a/translations/ja-JP/content/github/building-a-strong-community/locking-conversations.md b/translations/ja-JP/content/github/building-a-strong-community/locking-conversations.md
index 9c72983f614a..99c3442d8d88 100644
--- a/translations/ja-JP/content/github/building-a-strong-community/locking-conversations.md
+++ b/translations/ja-JP/content/github/building-a-strong-community/locking-conversations.md
@@ -15,7 +15,7 @@ It's appropriate to lock a conversation when the entire conversation is not cons

-会話がロックされている間も、[書き込みアクセスを持つユーザ](/articles/repository-permission-levels-for-an-organization/)と[リポジトリのオーナーおよびコラボレーター](/articles/permission-levels-for-a-user-account-repository/#collaborator-access-on-a-repository-owned-by-a-user-account)はコメントを追加または削除したり、非表示にしたりできます。
+会話がロックされている間も、[書き込みアクセスを持つユーザ](/articles/repository-permission-levels-for-an-organization/)と[リポジトリのオーナーおよびコラボレーター](/articles/permission-levels-for-a-user-account-repository/#collaborator-access-for-a-repository-owned-by-a-user-account)はコメントを追加または削除したり、非表示にしたりできます。
アーカイブされていないリポジトリでロックされた会話を検索するには、検索修飾子 `is:locked` および `archived:false` を使用できます。 会話はアーカイブされたリポジトリで自動的にロックされます。 詳細は「[Issue およびプルリクエストを検索する](/articles/searching-issues-and-pull-requests#search-based-on-whether-a-conversation-is-locked)」を参照してください。
diff --git a/translations/ja-JP/content/github/building-a-strong-community/manually-creating-a-single-issue-template-for-your-repository.md b/translations/ja-JP/content/github/building-a-strong-community/manually-creating-a-single-issue-template-for-your-repository.md
index a6f8ba2fd626..f5eb288e50d7 100644
--- a/translations/ja-JP/content/github/building-a-strong-community/manually-creating-a-single-issue-template-for-your-repository.md
+++ b/translations/ja-JP/content/github/building-a-strong-community/manually-creating-a-single-issue-template-for-your-repository.md
@@ -39,8 +39,12 @@ assignees: octocat
{% endif %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
{% data reusables.repositories.default-issue-templates %}
+{% endif %}
+
### Issue テンプレートを追加する
{% data reusables.repositories.navigate-to-repo %}
diff --git a/translations/ja-JP/content/github/building-a-strong-community/setting-guidelines-for-repository-contributors.md b/translations/ja-JP/content/github/building-a-strong-community/setting-guidelines-for-repository-contributors.md
index 4fe2a33389e0..e5118cded339 100644
--- a/translations/ja-JP/content/github/building-a-strong-community/setting-guidelines-for-repository-contributors.md
+++ b/translations/ja-JP/content/github/building-a-strong-community/setting-guidelines-for-repository-contributors.md
@@ -20,7 +20,11 @@ versions:
オーナーおよびコントリビューターの双方にとって、コントリビューションガイドラインは、プルリクエストや Issue のリジェクトや再提出の手間を未然に軽減するための有効な手段です。
-You can create default contribution guidelines for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. 詳しい情報については「[デフォルトのコミュニティ健全性ファイルを作成する](/github/building-a-strong-community/creating-a-default-community-health-file)」を参照してください。
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+You can create default contribution guidelines for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. 詳しい情報については「[デフォルトのコミュニティ健全性ファイルを作成する](/github/building-a-strong-community/creating-a-default-community-health-file)」を参照してください。
+
+{% endif %}
{% tip %}
@@ -53,5 +57,5 @@ You can create default contribution guidelines for your organization{% if curren
### 参考リンク
- The Open Source Guides' section "[Starting an Open Source Project](https://opensource.guide/starting-a-project/)"{% if currentVersion == "free-pro-team@latest" %}
-- [{% data variables.product.prodname_learning %}]({% data variables.product.prodname_learning_link %}){% endif %}
-- [リポジトリへのライセンスの追加](/articles/adding-a-license-to-a-repository)
+- [{% data variables.product.prodname_learning %}]({% data variables.product.prodname_learning_link %}){% endif %}{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- "[Adding a license to a repository](/articles/adding-a-license-to-a-repository)"{% endif %}
diff --git a/translations/ja-JP/content/github/collaborating-with-issues-and-pull-requests/about-branches.md b/translations/ja-JP/content/github/collaborating-with-issues-and-pull-requests/about-branches.md
index 3068c132f55d..1a1a47b95ed6 100644
--- a/translations/ja-JP/content/github/collaborating-with-issues-and-pull-requests/about-branches.md
+++ b/translations/ja-JP/content/github/collaborating-with-issues-and-pull-requests/about-branches.md
@@ -23,7 +23,7 @@ Branches allow you to develop features, fix bugs, or safely experiment with new
### About the default branch
-{% data reusables.branches.new-repo-default-branch %} The default branch is the branch that {% data variables.product.prodname_dotcom %} displays when anyone visits your repository. The default branch is also the initial branch that Git checks out locally out when someone clones the repository. {% data reusables.branches.default-branch-automatically-base-branch %}
+{% data reusables.branches.new-repo-default-branch %} The default branch is the branch that {% data variables.product.prodname_dotcom %} displays when anyone visits your repository. The default branch is also the initial branch that Git checks out locally when someone clones the repository. {% data reusables.branches.default-branch-automatically-base-branch %}
By default, {% data variables.product.product_name %} names the default branch {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}`main`{% else %}`master`{% endif %} in any new repository.
@@ -75,7 +75,7 @@ By default, {% data variables.product.product_name %} names the default branch {
- ブランチでプルリクエストレビュー必須が有効化されている場合、プルリクエストレビューポリシー中のすべての要求が満たされるまでは、ブランチに変更をマージできません。 詳しい情報については[プルリクエストのマージ](/articles/merging-a-pull-request)を参照してください。
- ブランチでコードオーナーからの必須レビューが有効化されており、プルリクエストがオーナーを持つコードを変更している場合、コードオーナーがプルリクエストを承認しなければ、そのプルリクエストはマージできません。 詳細は「[コードオーナーについて](/articles/about-code-owners)」を参照してください。
- ブランチでコミット署名必須が有効化されている場合、署名および検証されていないコミットはブランチにプッシュできません。 For more information, see "[About commit signature verification](/articles/about-commit-signature-verification)" and "[About required commit signing](/articles/about-required-commit-signing)."{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
-- {% data variables.product.prodname_dotcom %} のコンフリクトエディターを使用して、保護されたブランチから作成したプルリクエストのコンフリクトを修正する場合、{% data variables.product.prodname_dotcom %} はプルリクエストの代替ブランチを作成して、コンフリクトの解決をマージできるようにします。 詳しい情報については、「[{% data variables.product.prodname_dotcom %} でマージコンフリクトを解決する](/github/collaborating-with-issues-and-pull-requests/resolving-a-merge-conflict-on-github)」を参照してください。{% endif %}
+- If you use {% data variables.product.prodname_dotcom %}'s conflict editor to fix conflicts for a pull request that you created from a protected branch, {% data variables.product.prodname_dotcom %} helps you to create an alternative branch for the pull request, so that your resolution of the conflicts can be merged. 詳しい情報については、「[{% data variables.product.prodname_dotcom %} でマージコンフリクトを解決する](/github/collaborating-with-issues-and-pull-requests/resolving-a-merge-conflict-on-github)」を参照してください。{% endif %}
### 参考リンク
diff --git a/translations/ja-JP/content/github/collaborating-with-issues-and-pull-requests/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility.md b/translations/ja-JP/content/github/collaborating-with-issues-and-pull-requests/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility.md
index 7383d7a7f259..fe2e84743195 100644
--- a/translations/ja-JP/content/github/collaborating-with-issues-and-pull-requests/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility.md
+++ b/translations/ja-JP/content/github/collaborating-with-issues-and-pull-requests/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility.md
@@ -16,14 +16,20 @@ versions:
プライベートリポジトリを削除すると、そのプライベートフォークもすべて削除されます。
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
#### パブリックリポジトリを削除する
パブリックリポジトリを削除すると、既存のパブリックフォークの 1 つが新しい親リポジトリとして選択されます。 他のすべてのリポジトリはこの新しい親から分岐し、その後のプルリクエストはこの新しい親に送られます。
+{% endif %}
+
#### プライベートフォークと権限
{% data reusables.repositories.private_forks_inherit_permissions %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
#### パブリックリポジトリをプライベートリポジトリに変更する
パブリックリポジトリを非公開にすると、そのパブリックフォークは新しいネットワークに分割されます。 パブリックリポジトリの削除と同様に、既存のパブリックフォークの 1 つが新しい親リポジトリとして選択され、他のすべてのリポジトリはこの新しい親から分岐されます。 後続のプルリクエストは、この新しい親に行きます。
@@ -46,9 +52,30 @@ versions:
プライベートリポジトリを公開してから削除しても、そのプライベートフォークは独立したプライベートリポジトリとして別々のネットワークに存在し続けます。
+{% endif %}
+
+{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %}
+
+#### Changing the visibility of an internal repository
+
+{% note %}
+
+**Note:** {% data reusables.gated-features.internal-repos %}
+
+{% endnote %}
+
+If the policy for your enterprise permits forking, any fork of an internal repository will be private. If you change the visibility of an internal repository, any fork owned by an organization or user account will remain private.
+
+##### Deleting the internal repository
+
+If you change the visibility of an internal repository and then delete the repository, the forks will continue to exist in a separate network.
+
+{% endif %}
+
### 参考リンク
- 「[リポジトリの可視性を設定する](/articles/setting-repository-visibility)」
- [フォークについて](/articles/about-forks)
- 「[リポジトリのフォークポリシーを管理する](/github/administering-a-repository/managing-the-forking-policy-for-your-repository)」
- 「[Organization のフォークポリシーを管理する](/github/setting-up-and-managing-organizations-and-teams/managing-the-forking-policy-for-your-organization)」
+- "{% if currentVersion == "free-pro-team@latest" %}[Enforcing repository management policies in your enterprise account](/github/setting-up-and-managing-your-enterprise/enforcing-repository-management-policies-in-your-enterprise-account#enforcing-a-policy-on-forking-private-or-internal-repositories){% else %}[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#enforcing-a-policy-on-forking-private-or-internal-repositories){% endif %}"
diff --git a/translations/ja-JP/content/github/creating-cloning-and-archiving-repositories/about-readmes.md b/translations/ja-JP/content/github/creating-cloning-and-archiving-repositories/about-readmes.md
index beb733a866a0..e6ae03cd1a83 100644
--- a/translations/ja-JP/content/github/creating-cloning-and-archiving-repositories/about-readmes.md
+++ b/translations/ja-JP/content/github/creating-cloning-and-archiving-repositories/about-readmes.md
@@ -11,7 +11,11 @@ versions:
github-ae: '*'
---
-A README file, along with {% if currentVersion == "free-pro-team@latest" %}a [repository license](/articles/licensing-a-repository), [contribution guidelines](/articles/setting-guidelines-for-repository-contributors), and a [code of conduct](/articles/adding-a-code-of-conduct-to-your-project){% else %}a [repository license](/articles/licensing-a-repository) and [contribution guidelines](/articles/setting-guidelines-for-repository-contributors){% endif %}, helps you communicate expectations for and manage contributions to your project.
+### READMEについて
+
+You can add a README file to a repository to communicate important information about your project. A README, along with a repository license{% if currentVersion == "free-pro-team@latest" %}, contribution guidelines, and a code of conduct{% elsif enterpriseServerVersions contains currentVersion %} and contribution guidelines{% endif %}, communicates expectations for your project and helps you manage contributions.
+
+For more information about providing guidelines for your project, see {% if currentVersion == "free-pro-team@latest" %}"[Adding a code of conduct to your project](/github/building-a-strong-community/adding-a-code-of-conduct-to-your-project)" and {% endif %}"[Setting up your project for healthy contributions](/github/building-a-strong-community/setting-up-your-project-for-healthy-contributions)."
多くの場合、READMEはリポジトリへの訪問者が最初に目にするアイテムです。 通常、README ファイルには以下の情報が含まれています:
- このプロジェクトが行うこと
@@ -26,8 +30,12 @@ README ファイルをリポジトリのルート、`docs`、または隠れデ
{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21"%}
+
{% data reusables.profile.profile-readme %}
+{% endif %}
+

{% endif %}
diff --git a/translations/ja-JP/content/github/creating-cloning-and-archiving-repositories/licensing-a-repository.md b/translations/ja-JP/content/github/creating-cloning-and-archiving-repositories/licensing-a-repository.md
index 2f21c389290c..df64df6c88e0 100644
--- a/translations/ja-JP/content/github/creating-cloning-and-archiving-repositories/licensing-a-repository.md
+++ b/translations/ja-JP/content/github/creating-cloning-and-archiving-repositories/licensing-a-repository.md
@@ -7,7 +7,6 @@ redirect_from:
versions:
free-pro-team: '*'
enterprise-server: '*'
- github-ae: '*'
---
### 適切なライセンスを選択する
@@ -18,7 +17,7 @@ versions:
{% note %}
-**Note:** If you publish your source code in a public repository on GitHub, {% if currentVersion == "free-pro-team@latest" %}according to the [Terms of Service](/articles/github-terms-of-service), {% endif %}other GitHub users have the right to view and fork your repository within the GitHub site. すでにパブリックリポジトリを作成しており、ユーザによるアクセスを禁止したい場合には、リポジトリをプライベートにすることができます。 パブリックリポジトリをプライベートリポジトリに変換しても、他のユーザが作成した既存のフォークやローカル コピーは存続します。 詳細は「[パブリックリポジトリをプライベートにする](/articles/making-a-public-repository-private)」を参照してください。
+**Note:** If you publish your source code in a public repository on {% data variables.product.product_name %}, {% if currentVersion == "free-pro-team@latest" %}according to the [Terms of Service](/articles/github-terms-of-service), {% endif %}other users of {% data variables.product.product_location %} have the right to view and fork your repository. If you have already created a repository and no longer want users to have access to the repository, you can make the repository private. When you change the visibility of a repository to private, existing forks or local copies created by other users will still exist. 詳細は「[リポジトリの可視性を設定する](/github/administering-a-repository/setting-repository-visibility)」を参照してください。
{% endnote %}
diff --git a/translations/ja-JP/content/github/developing-online-with-codespaces/using-codespaces-in-visual-studio.md b/translations/ja-JP/content/github/developing-online-with-codespaces/using-codespaces-in-visual-studio.md
index 8115b7d41243..bb4fd7472e62 100644
--- a/translations/ja-JP/content/github/developing-online-with-codespaces/using-codespaces-in-visual-studio.md
+++ b/translations/ja-JP/content/github/developing-online-with-codespaces/using-codespaces-in-visual-studio.md
@@ -46,8 +46,12 @@ Connecting to {% data variables.product.prodname_github_codespaces %} with the
### Configuring a codespace for {% data variables.product.prodname_vs %}
-The default codespace environment created by {% data variables.product.prodname_vs %} includes popular frameworks and tools such as .NET Core, Microsoft SQL Server, Python, and the Windows SDK. {% data variables.product.prodname_github_codespaces %} created with {% data variables.product.prodname_vs %} can be customized through a subset of `devcontainers.json` properties and a new tool called devinit, included with {% data variables.product.prodname_vs %}.
+A codespace, created with {% data variables.product.prodname_vs %}, can be customized through a new tool called devinit, a command line tool included with {% data variables.product.prodname_vs %}.
#### devinit
-The [devinit](https://docs.microsoft.com/visualstudio/devinit/getting-started-with-devinit) command-line tool lets you install additional frameworks and tools into your Windows development codespaces, as well as run PowerShell scripts or modify environment variables. devinit supports a configuration file called [devinit.json](https://docs.microsoft.com/visualstudio/devinit/devinit-json), which can be added to your project for creating customized and repeatable development environments. For more information about Windows codespace configuration and devinit, see [Customize a codespace](https://docs.microsoft.com/visualstudio/ide/codespaces/customize-codespaces) in the {% data variables.product.prodname_vs %} documentation.
+[devinit](https://docs.microsoft.com/visualstudio/devinit/getting-started-with-devinit) lets you install additional frameworks and tools into your Windows development codespaces, modify environment variables, and more.
+
+devinit supports a configuration file called [devinit.json](https://docs.microsoft.com/visualstudio/devinit/devinit-json). You can add this file to your project if you want to create a customized and repeatable development environment. When you use devinit with a [devcontainer.json](https://docs.microsoft.com/visualstudio/ide/codespaces/customize-codespaces#running-devinit-when-creating-a-codespace) file, your codespaces will be automatically configured on creation.
+
+For more information about Windows codespace configuration and devinit, see [Customize a codespace](https://docs.microsoft.com/visualstudio/ide/codespaces/customize-codespaces) in the {% data variables.product.prodname_vs %} documentation. For more information about devinit, see [Getting started with devinit](https://docs.microsoft.com/visualstudio/devinit/getting-started-with-devinit).
diff --git a/translations/ja-JP/content/github/getting-started-with-github/create-a-repo.md b/translations/ja-JP/content/github/getting-started-with-github/create-a-repo.md
index d30316adaffd..2b835092e767 100644
--- a/translations/ja-JP/content/github/getting-started-with-github/create-a-repo.md
+++ b/translations/ja-JP/content/github/getting-started-with-github/create-a-repo.md
@@ -10,14 +10,26 @@ versions:
github-ae: '*'
---
+{% if currentVersion == "free-pro-team@latest" %}
+
オープンソースプロジェクトを含む、さまざまなプロジェクトを {% data variables.product.product_name %} リポジトリに保存できます。 [オープンソースプロジェクト](http://opensource.org/about)では、より優れた信頼性のあるソフトウェアを作成するためにコードを共有できます。
+{% elsif enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
+
+You can store a variety of projects in {% data variables.product.product_name %} repositories, including innersource projects. With innersource, you can share code to make better, more reliable software. For more information on innersource, see {% data variables.product.company_short %}'s whitepaper "[An introduction to innersource](https://resources.github.com/whitepapers/introduction-to-innersource/)."
+
+{% endif %}
+
+{% if currentVersion == "free-pro-team@latest" %}
+
{% note %}
**メモ:** オープンソースプロジェクトのパブリックリポジトリを作成できます。 パブリックリポジトリを作成する際は、他のユーザにどのようにプロジェクトを共有してほしいのかを定義する[ライセンスファイル](http://choosealicense.com/)を含めるようにしてください。 {% data reusables.open-source.open-source-guide-repositories %} {% data reusables.open-source.open-source-learning-lab %}
{% endnote %}
+{% endif %}
+
{% data reusables.repositories.create_new %}
2. リポジトリに、短くて覚えやすい名前を入力します。 たとえば、"hello-world" といった名前です。 
3. 必要な場合、リポジトリの説明を追加します。 For example, "My first repository on
diff --git a/translations/ja-JP/content/github/getting-started-with-github/fork-a-repo.md b/translations/ja-JP/content/github/getting-started-with-github/fork-a-repo.md
index fe37d15bedda..20c6a8f76c9d 100644
--- a/translations/ja-JP/content/github/getting-started-with-github/fork-a-repo.md
+++ b/translations/ja-JP/content/github/getting-started-with-github/fork-a-repo.md
@@ -25,10 +25,16 @@ versions:
オープンソースソフトウェアは、コードを共有することで、より優れた、より信頼性の高いソフトウェアを作成可能にするという考えに基づいています。 詳しい情報については、Open Source Initiative の「[Open Source Initiative について](http://opensource.org/about)」を参照してください。
+For more information about applying open source principles to your organization's development work on {% data variables.product.product_location %}, see {% data variables.product.prodname_dotcom %}'s whitepaper "[An introduction to innersource](https://resources.github.com/whitepapers/introduction-to-innersource/)."
+
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
他のユーザのプロジェクトのフォークからパブリックリポジトリを作成する際は、プロジェクトの他者との共有方法を定義するライセンスファイルを必ず含めてください。 詳しい情報については、choosealicense の「[オープンソースのライセンスを選択する](http://choosealicense.com/)」を参照してください。
{% data reusables.open-source.open-source-guide-repositories %} {% data reusables.open-source.open-source-learning-lab %}
+{% endif %}
+
{% note %}
**注釈**: {% data reusables.repositories.desktop-fork %}
diff --git a/translations/ja-JP/content/github/getting-started-with-github/setting-up-a-trial-of-github-enterprise-server.md b/translations/ja-JP/content/github/getting-started-with-github/setting-up-a-trial-of-github-enterprise-server.md
index 4a6f8c24857b..480050dc0030 100644
--- a/translations/ja-JP/content/github/getting-started-with-github/setting-up-a-trial-of-github-enterprise-server.md
+++ b/translations/ja-JP/content/github/getting-started-with-github/setting-up-a-trial-of-github-enterprise-server.md
@@ -36,7 +36,7 @@ versions:
- [Quick start guide to {% data variables.product.prodname_dotcom %}](https://resources.github.com/webcasts/Quick-start-guide-to-GitHub/) ウェブキャスト
- {% data variables.product.prodname_dotcom %} ガイドの [Understanding the {% data variables.product.prodname_dotcom %}flow](https://guides.github.com/introduction/flow/)
- {% data variables.product.prodname_dotcom %} ガイドの [Hello World](https://guides.github.com/activities/hello-world/)
-3. To configure your instance to meet your organization's needs, see "[Configuring your enterprise](/admin/configuration/configuring-your-enterprise)."
+3. To configure your instance to meet your organization's needs, see "[Configuring your enterprise](/enterprise/admin/configuration/configuring-your-enterprise)."
4. {% data variables.product.prodname_ghe_server %} とご使用のアイデンティティプロバイダとを統合するには、「[SAML を使用する](/enterprise/admin/user-management/using-saml)」および「[LDAP を使用する](/enterprise/admin/authentication/using-ldap)」を参照してください。
5. 個人をトライアルに招待します。人数制限はありません。
- ビルトイン認証または設定済みアイデンティティプロバイダを使用して、ユーザを {% data variables.product.prodname_ghe_server %} インスタンスに追加します。 詳細は「[ビルトイン認証を使用する](/enterprise/admin/user-management/using-built-in-authentication)」を参照してください。
diff --git a/translations/ja-JP/content/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies.md b/translations/ja-JP/content/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies.md
index c0f46c36576b..a628b146fd7f 100644
--- a/translations/ja-JP/content/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies.md
+++ b/translations/ja-JP/content/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies.md
@@ -71,7 +71,7 @@ When {% data variables.product.product_name %} identifies a vulnerable dependenc
You can see all of the alerts that affect a particular project{% if currentVersion == "free-pro-team@latest" %} on the repository's Security tab or{% endif %} in the repository's dependency graph.{% if currentVersion == "free-pro-team@latest" %} For more information, see "[Viewing and updating vulnerable dependencies in your repository](/articles/viewing-and-updating-vulnerable-dependencies-in-your-repository)."{% endif %}
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
-By default, we notify people with admin permissions in the affected repositories about new {% data variables.product.prodname_dependabot_alerts %}.{% endif %} {% if currentVersion == "free-pro-team@latest" %}{% data variables.product.product_name %} never publicly discloses identified vulnerabilities for any repository. You can also make {% data variables.product.prodname_dependabot_alerts %} visible to additional people or teams working repositories that you own or have admin permissions for. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-dependabot-alerts)."
+By default, we notify people with admin permissions in the affected repositories about new {% data variables.product.prodname_dependabot_alerts %}.{% endif %} {% if currentVersion == "free-pro-team@latest" %}{% data variables.product.product_name %} never publicly discloses identified vulnerabilities for any repository. You can also make {% data variables.product.prodname_dependabot_alerts %} visible to additional people or teams working repositories that you own or have admin permissions for. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-security-alerts)."
{% endif %}
{% if enterpriseServerVersions contains currentVersion and currentVersion ver_lt "enterprise-server@2.22" %}
diff --git a/translations/ja-JP/content/github/managing-subscriptions-and-notifications-on-github/about-notifications.md b/translations/ja-JP/content/github/managing-subscriptions-and-notifications-on-github/about-notifications.md
index cda48aa1504f..7991804afa6f 100644
--- a/translations/ja-JP/content/github/managing-subscriptions-and-notifications-on-github/about-notifications.md
+++ b/translations/ja-JP/content/github/managing-subscriptions-and-notifications-on-github/about-notifications.md
@@ -21,7 +21,12 @@ versions:
- 特定の Issue、プルリクエスト、または Gist の会話。
- リポジトリまたは Team ディスカッション内のすべてのアクティビティ。
- {% data variables.product.prodname_actions %} で設定されたリポジトリ内のワークフローのステータスなどの CI アクティビティ。
+{% if currentVersion == "free-pro-team@latest" %}
+- Issues, pulls requests, releases and discussions (if enabled) in a repository.
+{% endif %}
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}
- リポジトリ内のリリース。
+{% endif %}
フォークを除き、あなたがプッシュアクセスを持つすべてのリポジトリを自動的にWatchすることもできます。 [**Watch**] をクリックすると、手動でアクセスできる他のリポジトリを Watch できます。
diff --git a/translations/ja-JP/content/github/managing-subscriptions-and-notifications-on-github/configuring-notifications.md b/translations/ja-JP/content/github/managing-subscriptions-and-notifications-on-github/configuring-notifications.md
index f9f96cfcf172..74287c9c48c1 100644
--- a/translations/ja-JP/content/github/managing-subscriptions-and-notifications-on-github/configuring-notifications.md
+++ b/translations/ja-JP/content/github/managing-subscriptions-and-notifications-on-github/configuring-notifications.md
@@ -21,16 +21,17 @@ versions:
### 通知配信オプション
-You have three basic options for notification delivery:
- - the notifications inbox on {% data variables.product.product_name %}{% if currentVersion == "free-pro-team@latest" %}
- - {% data variables.product.product_name %}{% endif %} のインボックスと同期する {% data variables.product.prodname_mobile %} の通知インボックス
- - an email client that uses a verified email address, which can also sync with the notifications inbox on {% data variables.product.product_name %}{% if currentVersion == "free-pro-team@latest" %} and {% data variables.product.prodname_mobile %}{% endif %}
+You can receive notifications for activity on {% data variables.product.product_name %} in the following locations.
+
+ - The notifications inbox in the {% data variables.product.product_name %} web interface{% if currentVersion == "free-pro-team@latest" %}
+ - The notifications inbox on {% data variables.product.prodname_mobile %}, which syncs with the inbox on {% data variables.product.product_name %}{% endif %}
+ - An email client that uses a verified email address, which can also sync with the notifications inbox on {% data variables.product.product_name %}{% if currentVersion == "free-pro-team@latest" %} and {% data variables.product.prodname_mobile %}{% endif %}
{% if currentVersion == "free-pro-team@latest" %}
{% data reusables.notifications-v2.notifications-inbox-required-setting %} For more information, see "[Choosing your notification settings](#choosing-your-notification-settings)."
{% endif %}
-{% data reusables.notifications-v2.tip-for-syncing-email-and-your-inbox-on-github %}
+{% data reusables.notifications.shared_state %}
#### 通知インボックスの利点
@@ -59,8 +60,21 @@ Email notifications also allow flexibility with the types of notifications you r
### 参加と Watch 対象の通知について
-When you watch a repository, you're subscribing to updates for activity in that repository. Similarly, when you watch a specific team's discussions, you're subscribing to all conversation updates on that team's page. To see repositories that you're watching, see [https://github.com/watching](https://github.com/watching). 詳しい情報については「[GitHub上でのサブスクリプションと通知の管理](/github/managing-subscriptions-and-notifications-on-github/managing-subscriptions-for-activity-on-github)」を参照してください。
+When you watch a repository, you're subscribing to updates for activity in that repository. Similarly, when you watch a specific team's discussions, you're subscribing to all conversation updates on that team's page. 詳しい情報については[Team ディスカッションについて](/github/building-a-strong-community/about-team-discussions)を参照してください。
+
+To see repositories that you're watching, go to your [watching page](https://github.com/watching). 詳しい情報については「[GitHub上でのサブスクリプションと通知の管理](/github/managing-subscriptions-and-notifications-on-github/managing-subscriptions-for-activity-on-github)」を参照してください。
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}
+#### 通知を設定する
+{% endif %}
+You can configure notifications for a repository on the repository page, or on your watching page.
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %} You can choose to only receive notifications for releases in a repository, or ignore all notifications for a repository.{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+
+#### About custom notifications
+{% data reusables.notifications-v2.custom-notifications-beta %}
+You can customize notifications for a repository, for example, you can choose to only be notified when updates to one or more types of events (issues, pull request, releases, discussions) happen within a repository, or ignore all notifications for a repository.
+{% endif %} For more information, see "[Viewing your subscriptions](/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions#configuring-your-watch-settings-for-an-individual-repository)."
+#### Participating in conversations
Anytime you comment in a conversation or when someone @mentions your username, you are _participating_ in a conversation. By default, you are automatically subscribed to a conversation when you participate in it. You can unsubscribe from a conversation you've participated in manually by clicking **Unsubscribe** on the issue or pull request or through the **Unsubscribe** option in the notifications inbox.
For conversations you're watching or participating in, you can choose whether you want to receive notifications by email or through the notifications inbox on {% data variables.product.product_name %}{% if currentVersion == "free-pro-team@latest" %} and {% data variables.product.prodname_mobile %}{% endif %}.
@@ -95,7 +109,9 @@ Choose a default email address where you want to send updates for conversations
- プルリクエストのプッシュ。
- Issue やプルリクエストのオープン、コメント、クローズなどの、自分自身の操作による更新。
-Depending on the organization that owns the repository, you can also send notifications to different email addresses for specific repositories. For example, you can send notifications for a specific public repository to a verified personal email address. Your organization may require the email address to be verified for a specific domain. 詳しい情報については、「[Organization のメール通知の送信先を選択する](/github/managing-subscriptions-and-notifications-on-github/configuring-notifications#choosing-where-your-organizations-email-notifications-are-sent)」を参照してください。
+Depending on the organization that owns the repository, you can also send notifications to different email addresses. Your organization may require the email address to be verified for a specific domain. For more information, see "[Choosing where your organization’s email notifications are sent](/github/managing-subscriptions-and-notifications-on-github/configuring-notifications#choosing-where-your-organizations-email-notifications-are-sent)."
+
+You can also send notifications for a specific repository to an email address. For more information, see "[About email notifications for pushes to your repository](/github/administering-a-repository/about-email-notifications-for-pushes-to-your-repository)."
{% data reusables.notifications-v2.email-notification-caveats %}
diff --git a/translations/ja-JP/content/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions.md b/translations/ja-JP/content/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions.md
index 906b39ae2cb1..9bb6ca44db2f 100644
--- a/translations/ja-JP/content/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions.md
+++ b/translations/ja-JP/content/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions.md
@@ -55,6 +55,13 @@ When you unwatch a repository, you unsubscribe from future updates from that rep
{% data reusables.notifications.access_notifications %}
1. 左側のサイドバーの、リポジトリリストの下にある [Manage notifications] ドロップダウンを使用して、[**Watched repositories**] をクリックします。 ![[Manage notifications] ドロップダウンメニューオプション](/assets/images/help/notifications-v2/manage-notifications-options.png)
2. Watch しているリポジトリのページで、Watchしているリポジトリを評価した後、次のいずれかを選択します。
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}
- リポジトリの Watch 解除
- リポジトリのリリースのみを Watch
- リポジトリのすべての通知を無視
+{% endif %}
+{% if currentVersion == "free-pro-team@latest" %}
+ - リポジトリの Watch 解除
+ - リポジトリのすべての通知を無視
+ - customize the types of event you receive notifications for (issues, pull requests, releases or discussions, if enabled)
+{% endif %}
\ No newline at end of file
diff --git a/translations/ja-JP/content/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions.md b/translations/ja-JP/content/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions.md
index 5ff0b00e17e9..b3965a51ab73 100644
--- a/translations/ja-JP/content/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions.md
+++ b/translations/ja-JP/content/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions.md
@@ -32,7 +32,16 @@ When your inbox has too many notifications to manage, consider whether you have
詳しい情報については、「[通知を設定する](/github/managing-subscriptions-and-notifications-on-github/configuring-notifications#automatic-watching)」を参照してください。
-To see an overview of your repository subscriptions, see "[Reviewing repositories that you're watching](#reviewing-repositories-that-youre-watching)." Many people forget about repositories that they've chosen to watch in the past. From the "Watched repositories" page you can quickly unwatch repositories. For more information on ways to unsubscribe, see "[Managing subscriptions](/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions)."
+To see an overview of your repository subscriptions, see "[Reviewing repositories that you're watching](#reviewing-repositories-that-youre-watching)."
+{% if currentVersion == "free-pro-team@latest" %}
+{% tip %}
+
+**Tip:** You can select the types of event to be notified of by using the **Custom** option of the **Watch/Unwatch** dropdown list in your [watching page](https://github.com/watching) or on any repository page on {% data variables.product.prodname_dotcom_the_website %}. For more information, see "[Configuring your watch settings for an individual repository](#configuring-your-watch-settings-for-an-individual-repository)" below.
+
+{% endtip %}
+{% endif %}
+
+Many people forget about repositories that they've chosen to watch in the past. From the "Watched repositories" page you can quickly unwatch repositories. For more information on ways to unsubscribe, see "[Unwatch recommendations](https://github.blog/changelog/2020-11-10-unwatch-recommendations/)" on {% data variables.product.prodname_blog %} and "[Managing your subscriptions](/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions)." You can also create a triage worflow to help with the notifications you receive. For guidance on triage workflows, see "[Customizing a workflow for triaging your notifications](/github/managing-subscriptions-and-notifications-on-github/customizing-a-workflow-for-triaging-your-notifications)."
### サブスクリプションのリストを確認する
@@ -55,20 +64,38 @@ To see an overview of your repository subscriptions, see "[Reviewing repositorie
### Watch しているリポジトリを確認する
1. 左側のサイドバーの、リポジトリリストの下にある [Manage notifications] ドロップダウンメニューを使用して、[**Watched repositories**] をクリックします。 ![[Manage notifications] ドロップダウンメニューオプション](/assets/images/help/notifications-v2/manage-notifications-options.png)
-
-3. Watch しているリポジトリを評価し、それらの更新がまだ関連していて有用であるかどうかを判断します。 リポジトリを Watch すると、そのリポジトリのすべての会話が通知されます。
-
+2. Watch しているリポジトリを評価し、それらの更新がまだ関連していて有用であるかどうかを判断します。 リポジトリを Watch すると、そのリポジトリのすべての会話が通知されます。
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}

+{% endif %}
+{% if currentVersion == "free-pro-team@latest" %}
+ 
+{% endif %}
{% tip %}
- **ヒント:** リポジトリを Watch する代わりに、リポジトリのリリースのみを Watch するか、リポジトリを完全に Watch 解除することを検討してください。 リポジトリを Watch 解除しても、@メンションされたときやスレッドに参加しているときには通知を受信することができます。 リポジトリのリリースのみを Watch する場合は、新しいリリースがあるとき、スレッドに参加しているとき、または自分または参加している Team が@メンションされたときにのみ通知されます。
+ **Tip:** Instead of watching a repository, consider only receiving notifications {% if currentVersion == "free-pro-team@latest" %}when there are updates to issues, pull requests, releases or discussions (if enabled for the repository), or any combination of these options,{% else %}for releases in a repository,{% endif %} or completely unwatching a repository.
+
+ リポジトリを Watch 解除しても、@メンションされたときやスレッドに参加しているときには通知を受信することができます。 When you configure to receive notifications for certain event types, you're only notified when there are updates to these event types in the repository, you're participating in a thread, or you or a team you're on is @mentioned.
{% endtip %}
### 個々のリポジトリの Watch 設定を行う
-リポジトリごとに Watch するどうかを選択できます。 また、新しいリリースのみの通知を受け取るか、リポジトリごとに無視するかどうかを選択することもできます。
+リポジトリごとに Watch するどうかを選択できます。 You can also choose to only be notified of {% if currentVersion == "free-pro-team@latest" %}certain event types such as issues, pull requests, discussions (if enabled for the repository) and {% endif %}new releases, or completely ignore an individual repository.
{% data reusables.repositories.navigate-to-repo %}
-2. 右上隅の [Watch] ドロップダウンメニューをクリックして、Watch オプションを選択します。 
+2. 右上隅の [Watch] ドロップダウンメニューをクリックして、Watch オプションを選択します。
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}
+ 
+{% endif %}
+{% if currentVersion == "free-pro-team@latest" %}
+ 
+{% data reusables.notifications-v2.custom-notifications-beta %}
+The **Custom** option allows you to further customize notifications so that you're only notified when specific events happen in the repository, in addition to participating and @mentions.
+
+ 
+
+If you select "Issues", you will be notified about, and subscribed to, updates on every issue (including those that existed prior to you selecting this option) in the repository. If you're @mentioned in a pull request in this repository, you'll receive notifications for that too, and you'll be subscribed to updates on that specific pull request, in addition to being notified about issues.
+
+{% endif %}
diff --git a/translations/ja-JP/content/github/managing-your-work-on-github/about-automation-for-project-boards.md b/translations/ja-JP/content/github/managing-your-work-on-github/about-automation-for-project-boards.md
index 5b063105f90b..665a38b2d8e8 100644
--- a/translations/ja-JP/content/github/managing-your-work-on-github/about-automation-for-project-boards.md
+++ b/translations/ja-JP/content/github/managing-your-work-on-github/about-automation-for-project-boards.md
@@ -31,9 +31,9 @@ versions:
### プロジェクトの進捗の追跡
-You can track the progress on your project board. Cards in the "To do", "In progress", or "Done" columns count toward the overall project progress. {% data reusables.project-management.project-progress-locations %}
+進捗は、プロジェクトボードで追跡できます。 [To do]、[In progress]、または [Done] 列のカードの数は、プロジェクトの進捗全体にカウントされます。 {% data reusables.project-management.project-progress-locations %}
-For more information, see "[Tracking progress on your project board](/github/managing-your-work-on-github/tracking-progress-on-your-project-board)."
+詳細については「[プロジェクトボードで進捗を追跡する](/github/managing-your-work-on-github/tracking-progress-on-your-project-board)」を参照してください。
### 参考リンク
- [プロジェクトボードの自動化を設定する](/articles/configuring-automation-for-project-boards){% if currentVersion == "free-pro-team@latest" %}
diff --git a/translations/ja-JP/content/github/managing-your-work-on-github/creating-a-label.md b/translations/ja-JP/content/github/managing-your-work-on-github/creating-a-label.md
index c9a654697f09..7ee83dc961ef 100644
--- a/translations/ja-JP/content/github/managing-your-work-on-github/creating-a-label.md
+++ b/translations/ja-JP/content/github/managing-your-work-on-github/creating-a-label.md
@@ -30,6 +30,6 @@ versions:
- [ラベルについて](/articles/about-labels)
- "[Issue およびプルリクエストにラベルを適用する](/articles/applying-labels-to-issues-and-pull-requests)"
- "[ラベルの編集](/articles/editing-a-label)"
-- "[Filtering issues and pull requests by labels](/articles/filtering-issues-and-pull-requests-by-labels)"{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "github-ae@latest" %}
+- [Issue およびプルリクエストをラベルでフィルタリングする](/articles/filtering-issues-and-pull-requests-by-labels){% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "github-ae@latest" %}
- [Organization 内のリポジトリのためのデフォルトラベルを管理する](/articles/managing-default-labels-for-repositories-in-your-organization)
{% endif %}
diff --git a/translations/ja-JP/content/github/managing-your-work-on-github/creating-an-issue.md b/translations/ja-JP/content/github/managing-your-work-on-github/creating-an-issue.md
index 6a57bc02ff9b..2e498959eb43 100644
--- a/translations/ja-JP/content/github/managing-your-work-on-github/creating-an-issue.md
+++ b/translations/ja-JP/content/github/managing-your-work-on-github/creating-an-issue.md
@@ -1,6 +1,7 @@
---
title: Issue の作成
intro: 'Issue は、バグ、拡張、その他リクエストの追跡に使用できます。'
+permissions: '読み取り権限を持つユーザは、Issue が有効なときリポジトリに Issue を作成できます。'
redirect_from:
- /articles/creating-an-issue
versions:
@@ -9,8 +10,6 @@ versions:
github-ae: '*'
---
-{% data reusables.repositories.create-issue-in-public-repository %}
-
既存のプルリクエストのコードに基づく新しい Issue を開くことができます。 詳しい情報については「[コードから Issue を開く](/github/managing-your-work-on-github/opening-an-issue-from-code)」を参照してください。
Issue または Pull Requestレビューのコメントから新しい Issue を直接開くことができます。 詳しい情報については「[コメントからIssueを開く](/github/managing-your-work-on-github/opening-an-issue-from-a-comment)」を参照してください。
diff --git a/translations/ja-JP/content/github/managing-your-work-on-github/deleting-a-label.md b/translations/ja-JP/content/github/managing-your-work-on-github/deleting-a-label.md
index cf368d35d5ec..cf8c17136e56 100644
--- a/translations/ja-JP/content/github/managing-your-work-on-github/deleting-a-label.md
+++ b/translations/ja-JP/content/github/managing-your-work-on-github/deleting-a-label.md
@@ -19,6 +19,6 @@ versions:
### 参考リンク
- "[Issue およびプルリクエストにラベルを適用する](/articles/applying-labels-to-issues-and-pull-requests)"
-- "[Filtering issues and pull requests by labels](/articles/filtering-issues-and-pull-requests-by-labels)"{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "github-ae@latest" %}
+- [Issue およびプルリクエストをラベルでフィルタリングする](/articles/filtering-issues-and-pull-requests-by-labels){% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "github-ae@latest" %}
- [Organization 内のリポジトリのためのデフォルトラベルを管理する](/articles/managing-default-labels-for-repositories-in-your-organization)
{% endif %}
diff --git a/translations/ja-JP/content/github/managing-your-work-on-github/editing-a-label.md b/translations/ja-JP/content/github/managing-your-work-on-github/editing-a-label.md
index e5cb2e38d00a..29e1e6ea49ba 100644
--- a/translations/ja-JP/content/github/managing-your-work-on-github/editing-a-label.md
+++ b/translations/ja-JP/content/github/managing-your-work-on-github/editing-a-label.md
@@ -24,6 +24,6 @@ versions:
- "[ラベルの作成](/articles/creating-a-label)"
- "[ラベルの削除](/articles/deleting-a-label)"
- "[Issue およびプルリクエストにラベルを適用する](/articles/applying-labels-to-issues-and-pull-requests)"
-- "[Filtering issues and pull requests by labels](/articles/filtering-issues-and-pull-requests-by-labels)"{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "github-ae@latest" %}
+- [Issue およびプルリクエストをラベルでフィルタリングする](/articles/filtering-issues-and-pull-requests-by-labels){% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "github-ae@latest" %}
- [Organization 内のリポジトリのためのデフォルトラベルを管理する](/articles/managing-default-labels-for-repositories-in-your-organization)
{% endif %}
diff --git a/translations/ja-JP/content/github/managing-your-work-on-github/file-attachments-on-issues-and-pull-requests.md b/translations/ja-JP/content/github/managing-your-work-on-github/file-attachments-on-issues-and-pull-requests.md
index 6a58cc25f63c..eb2ca5f1b35f 100644
--- a/translations/ja-JP/content/github/managing-your-work-on-github/file-attachments-on-issues-and-pull-requests.md
+++ b/translations/ja-JP/content/github/managing-your-work-on-github/file-attachments-on-issues-and-pull-requests.md
@@ -22,7 +22,7 @@ Issue やプルリクエストの会話にファイルを添付するには、
{% tip %}
-**Tip:** In many browsers, you can copy-and-paste images directly into the box.
+**ヒント:** 多くのブラウザでは、画像をコピーして直接ボックスに貼り付けることができます。
{% endtip %}
diff --git a/translations/ja-JP/content/github/managing-your-work-on-github/filtering-cards-on-a-project-board.md b/translations/ja-JP/content/github/managing-your-work-on-github/filtering-cards-on-a-project-board.md
index ee50ab6572c1..f8ff6db8f94a 100644
--- a/translations/ja-JP/content/github/managing-your-work-on-github/filtering-cards-on-a-project-board.md
+++ b/translations/ja-JP/content/github/managing-your-work-on-github/filtering-cards-on-a-project-board.md
@@ -22,7 +22,7 @@ versions:
- `status:pending`、`status:success`、または `status:failure` を使用して、カードをチェックステータスでフィルタリングする
- `type:issue`、`type:pr`、または `type:note` を使用して、カードをタイプでフィルタリングする
- `is:open`、`is:closed`、または `is:merged`と、`is:issue`、`is:pr`、または `is:note` とを使用して、カードをステータスとタイプでフィルタリングする
-- Filter cards by issues that are linked to a pull request by a closing reference using `linked:pr`{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.20" or currentVersion == "github-ae@latest" %}
+- `linked:pr`を使用してクローズしているリファレンスによってプルリクエストにリンクされている Issue でカードをフィルタリングする{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.20" or currentVersion == "github-ae@latest" %}
- `repo:ORGANIZATION/REPOSITORY` を使用して、Organization 全体のプロジェクトボード内のリポジトリでカードをフィルタリングする{% endif %}
1. フィルタリングしたいカードが含まれるプロジェクトボードに移動します。
diff --git a/translations/ja-JP/content/github/managing-your-work-on-github/linking-a-pull-request-to-an-issue.md b/translations/ja-JP/content/github/managing-your-work-on-github/linking-a-pull-request-to-an-issue.md
index e7f6945d45c8..cdc6a9ff2fb1 100644
--- a/translations/ja-JP/content/github/managing-your-work-on-github/linking-a-pull-request-to-an-issue.md
+++ b/translations/ja-JP/content/github/managing-your-work-on-github/linking-a-pull-request-to-an-issue.md
@@ -1,6 +1,6 @@
---
title: プルリクエストをIssueにリンクする
-intro: 'You can link a pull request to an issue to show that a fix is in progress and to automatically close the issue when the pull request is merged.'
+intro: 'プルリクエストをIssueにリンクして、修正が進行中であることを示し、プルリクエストがマージされるときIssueを自動的にクローズすることができます。'
redirect_from:
- /articles/closing-issues-via-commit-message/
- /articles/closing-issues-via-commit-messages/
@@ -20,7 +20,7 @@ versions:
### リンクされたIssueとプルリクエストについて
-You can link an issue to a pull request {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.20" or currentVersion == "github-ae@latest" %}manually or {% endif %}using a supported keyword in the pull request description.
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.20" or currentVersion == "github-ae@latest" %}手動で、または{% endif %}プルリクエストの説明でサポートされているキーワードを使用して、Issueをプルリクエストにリンクすることができます。
プルリクエストが対処するIssueにそのプルリクエストにリンクすると、コラボレータは、誰かがそのIssueに取り組んでいることを確認できます。 {% if currentVersion ver_lt "enterprise-server@2.21" %}プルリクエストとIssueが別のリポジトリにある場合は、プルリクエストをマージするユーザーにIssueをクローズする権限もあれば、そのマージが実行された後で{% data variables.product.product_name %}にリンクが表示されます。{% endif %}
@@ -50,7 +50,7 @@ You can link an issue to a pull request {% if currentVersion == "free-pro-team@l
* fix
* fixes
* fixed
-* 解決
+* resolve
* resolves
* resolved
@@ -62,7 +62,7 @@ You can link an issue to a pull request {% if currentVersion == "free-pro-team@l
| Issueが別のリポジトリにある | *KEYWORD* *OWNER*/*REPOSITORY*#*ISSUE-NUMBER* | `Fixes octo-org/octo-repo#100` |
| 複数の Issue | Issueごとに完全な構文を使用 | `Resolves #10, resolves #123, resolves octo-org/octo-repo#100` |
-{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.20" or currentVersion == "github-ae@latest" %}Only manually linked pull requests can be manually unlinked. キーワードを使用してリンクしたIssueのリンクを解除するには、プルリクエストの説明を編集してそのキーワードを削除する必要があります。{% endif %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.20" or currentVersion == "github-ae@latest" %}手動でリンクを解除できるのは、手動でリンクされたプルリクエストだけです。 キーワードを使用してリンクしたIssueのリンクを解除するには、プルリクエストの説明を編集してそのキーワードを削除する必要があります。{% endif %}
クローズするキーワードは、コミットメッセージでも使用できます。 デフォルトブランチにコミットをマージするとIssueはクローズされますが、そのコミットを含むプルリクエストは、リンクされたプルリクエストとしてリストされません。
diff --git a/translations/ja-JP/content/github/managing-your-work-on-github/opening-an-issue-from-a-comment.md b/translations/ja-JP/content/github/managing-your-work-on-github/opening-an-issue-from-a-comment.md
index 2add02de7020..e7afdd5ffd20 100644
--- a/translations/ja-JP/content/github/managing-your-work-on-github/opening-an-issue-from-a-comment.md
+++ b/translations/ja-JP/content/github/managing-your-work-on-github/opening-an-issue-from-a-comment.md
@@ -1,6 +1,7 @@
---
title: コメントからIssueを開く
intro: Issueまたはプルリクエストの特定のコメントから新しいIssueを開くことができます。
+permissions: '読み取り権限を持つユーザは、Issue が有効なときリポジトリに Issue を作成できます。'
versions:
free-pro-team: '*'
enterprise-server: '*'
@@ -9,6 +10,8 @@ versions:
コメントから開いたIssueには、コメントの元の投稿場所を示すスニペットが含まれています。
+{% data reusables.repositories.administrators-can-disable-issues %}
+
1. 開きたいIssueがあるコメントに移動します。
2. そのコメントで、{% octicon "kebab-horizontal" aria-label="The horizontal kebab icon" %} をクリックします。 
diff --git a/translations/ja-JP/content/github/managing-your-work-on-github/opening-an-issue-from-code.md b/translations/ja-JP/content/github/managing-your-work-on-github/opening-an-issue-from-code.md
index 90137fa292ee..81c91af136b7 100644
--- a/translations/ja-JP/content/github/managing-your-work-on-github/opening-an-issue-from-code.md
+++ b/translations/ja-JP/content/github/managing-your-work-on-github/opening-an-issue-from-code.md
@@ -1,6 +1,7 @@
---
title: コードから Issue を開く
intro: コードの特定の行または複数の行から、ファイルまたはプルリクエストで Issue を開くことができます。
+permissions: '読み取り権限を持つユーザは、Issue が有効なときリポジトリに Issue を作成できます。'
redirect_from:
- /articles/opening-an-issue-from-code
versions:
@@ -13,7 +14,7 @@ versions:

-{% data reusables.repositories.create-issue-in-public-repository %}
+{% data reusables.repositories.administrators-can-disable-issues %}
{% data reusables.repositories.navigate-to-repo %}
2. Issue で参照したいコードを探します。
diff --git a/translations/ja-JP/content/github/managing-your-work-on-github/tracking-progress-on-your-project-board.md b/translations/ja-JP/content/github/managing-your-work-on-github/tracking-progress-on-your-project-board.md
index 1b097666b68d..216b63b9c5a8 100644
--- a/translations/ja-JP/content/github/managing-your-work-on-github/tracking-progress-on-your-project-board.md
+++ b/translations/ja-JP/content/github/managing-your-work-on-github/tracking-progress-on-your-project-board.md
@@ -1,6 +1,6 @@
---
title: プロジェクトボードで進捗を追跡する
-intro: You can see the overall progress of your project in a progress bar.
+intro: プロジェクトの進捗全体は、プログレスバーで確認できます。
redirect_from:
- /articles/tracking-progress-on-your-project-board
versions:
@@ -11,7 +11,7 @@ versions:
{% data reusables.project-management.project-progress-locations %}
-1. Navigate to the project board where you want to enable or disable project progress tracking.
+1. プロジェクトの進捗の追跡を有効化または無効化したいプロジェクトボードに移動します。
{% data reusables.project-management.click-menu %}
{% data reusables.project-management.click-edit-sidebar-menu-project-board %}
-4. Select or deselect **Track project progress**.
+4. [**Track project progress**] を選択または選択解除します。
diff --git a/translations/ja-JP/content/github/managing-your-work-on-github/transferring-an-issue-to-another-repository.md b/translations/ja-JP/content/github/managing-your-work-on-github/transferring-an-issue-to-another-repository.md
index 2ef9b313f1f7..400403e4bb3c 100644
--- a/translations/ja-JP/content/github/managing-your-work-on-github/transferring-an-issue-to-another-repository.md
+++ b/translations/ja-JP/content/github/managing-your-work-on-github/transferring-an-issue-to-another-repository.md
@@ -11,7 +11,7 @@ versions:
他のリポジトリにオープン Issue を移譲するには、Issue のあるリポジトリおよびその Issue の移譲先のリポジトリの書き込み権限が必要です。 詳細は「[Organization のためのリポジトリの権限レベル](/articles/repository-permission-levels-for-an-organization)」を参照してください。
-同じユーザまたは Organization アカウントが所有するリポジトリ間においてのみ、Issue を移譲できます。 プライベートリポジトリからパブリックリポジトリへは、Issue を移譲できません。
+Issue を移動できるのは、同じユーザーまたは Organization アカウントが所有しているリポジトリ間のみです。{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}プライベートリポジトリからパブリックリポジトリへ Issue を移譲することはできません。{% endif %}
Issueを委譲する場合、コメントとアサインされた人は保持されます。 Issue のラベルとマイルストーンは保持されません。 このIssueは、ユーザー所有または組織全体のプロジェクトボードにとどまり、リポジトリのプロジェクトボードから削除されます。 詳細は「[プロジェクトボードについて](/articles/about-project-boards)」を参照してください。
diff --git a/translations/ja-JP/content/github/managing-your-work-on-github/using-search-to-filter-issues-and-pull-requests.md b/translations/ja-JP/content/github/managing-your-work-on-github/using-search-to-filter-issues-and-pull-requests.md
index 8dd0ce8af04c..5d9fb1ac6b72 100644
--- a/translations/ja-JP/content/github/managing-your-work-on-github/using-search-to-filter-issues-and-pull-requests.md
+++ b/translations/ja-JP/content/github/managing-your-work-on-github/using-search-to-filter-issues-and-pull-requests.md
@@ -40,7 +40,7 @@ Issueについては、以下も検索に利用できます。
- レビュー担当者が変更を要求したプルリクエストのフィルタリング: `state:open type:pr review:changes_requested`
- [レビュー担当者](/articles/about-pull-request-reviews/)によるプルリクエストのフィルタリング: `state:open type:pr reviewed-by:octocat`
- [レビューを要求された](/articles/requesting-a-pull-request-review)特定のユーザーによるプルリクエストのフィルタリング: `state:open type:pr review-requested:octocat`
-- Filter pull requests by the team requested for review: `state:open type:pr team-review-requested:github/atom`{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.20" or currentVersion == "github-ae@latest" %}
+- レビューを要求されたチームによるプルリクエストのフィルタリング: `state:open type:pr team-review-requested:github/atom`{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.20" or currentVersion == "github-ae@latest" %}
- プルリクエストでクローズできるIssueにリンクされているプルリクエストのフィルタリング: `linked:issue`{% endif %}
### 参考リンク
diff --git a/translations/ja-JP/content/github/managing-your-work-on-github/viewing-all-of-your-issues-and-pull-requests.md b/translations/ja-JP/content/github/managing-your-work-on-github/viewing-all-of-your-issues-and-pull-requests.md
index 1f0874b1e6bb..6d6593c46cc0 100644
--- a/translations/ja-JP/content/github/managing-your-work-on-github/viewing-all-of-your-issues-and-pull-requests.md
+++ b/translations/ja-JP/content/github/managing-your-work-on-github/viewing-all-of-your-issues-and-pull-requests.md
@@ -16,4 +16,4 @@ Issue およびプルリクエストダッシュボードは、すべてのペ
### 参考リンク
-- {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.20" or currentVersion == "github-ae@latest" %}”[Viewing your subscriptions](/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions#reviewing-repositories-that-youre-watching){% else %}”[Listing the repositories you're watching](/github/receiving-notifications-about-activity-on-github/listing-the-repositories-youre-watching){% endif %}"
+- {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.20" or currentVersion == "github-ae@latest" %}[サブスクリプションを表示する](/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions#reviewing-repositories-that-youre-watching){% else %}[Watch しているリポジトリのリスト](/github/receiving-notifications-about-activity-on-github/listing-the-repositories-youre-watching){% endif %}
diff --git a/translations/ja-JP/content/github/searching-for-information-on-github/about-searching-on-github.md b/translations/ja-JP/content/github/searching-for-information-on-github/about-searching-on-github.md
index 65b8ea65003b..bdf36a07f40f 100644
--- a/translations/ja-JP/content/github/searching-for-information-on-github/about-searching-on-github.md
+++ b/translations/ja-JP/content/github/searching-for-information-on-github/about-searching-on-github.md
@@ -14,7 +14,7 @@ versions:
github-ae: '*'
---
-{% data variables.product.product_name %}全体にわたってグローバルに検索できます。あるいは、検索を特定のリポジトリや Organization に絞ることもできます。
+{% data reusables.search.you-can-search-globally %}
- {% data variables.product.product_name %} 全体にわたってグローバルに検索するには、探している内容を任意のページの上部にある検索フィールドに入力し、[All {% data variables.product.prodname_dotcom %}] を検索ドロップダウンメニューで選択します。
- 特定のリポジトリあるいは Organization 内で検索するには、そのリポジトリあるいは Organization のページにアクセスし、検索する内容をページの上部にある検索フィールドに入力し、**Enter** を押してください。
@@ -23,7 +23,8 @@ versions:
**ノート:**
-- {% data reusables.search.required_login %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- {% data reusables.search.required_login %}{% endif %}
- {% data variables.product.prodname_pages %}サイトは、{% data variables.product.product_name %}上では検索できません。 ただし、コンテンツのソースがリポジトリのデフォルトブランチにある場合は、コード検索を使って検索できます。 詳しい情報については[コードの検索](/articles/searching-code)を参照してください。 {% data variables.product.prodname_pages %}に関する詳しい情報については、[GitHub Pages とは何ですか? ](/articles/what-is-github-pages/)を参照してください。
- 現在、GitHub の検索は完全一致をサポートしていません。
- コードファイルのどこを検索しても、返されるのは各ファイルで最初の 2 つの結果のみです。
@@ -36,7 +37,7 @@ versions:
### {% data variables.product.prodname_dotcom %}での検索の種類
-以下の種類の情報が、すべてのパブリックな {% data variables.product.product_name %}のリポジトリ、およびアクセス権のあるすべてのプライベートな {% data variables.product.product_name %}のリポジトリにわたって検索できます。
+以下の情報は、{% data variables.product.product_location %} でアクセスできるすべてのリポジトリから検索できます。
- [リポジトリ](/articles/searching-for-repositories)
- [Topics](/articles/searching-topics)
@@ -63,9 +64,9 @@ versions:
双方の環境にわたる検索は、{% data variables.product.prodname_enterprise %} からしか行えません。 検索の範囲を環境で狭めるには、{% data variables.search.advanced_url %} 上のフィルタオプションを使うか、検索プレフィックス `environment:` を利用できます。 {% data variables.product.prodname_enterprise %} 上のコンテンツだけを検索するには、`environment:local` という検索構文を使います。 {% data variables.product.prodname_dotcom_the_website %} 上のコンテンツだけを検索するには`environment:github` を使います。
{% data variables.product.prodname_enterprise %} サイト管理者は、接続された {% data variables.product.prodname_ghe_cloud %} Organization 中のすべてのパブリックリポジトリ、すべてのプライベートリポジトリ、あるいは特定のプライベートリポジトリのみに対して {% data variables.product.prodname_unified_search %} を有効化できます。
-If your site administrator enables
+サイト管理者が
-{% data variables.product.prodname_unified_search %} in private repositories, you can only search in the private repositories that the administrator enabled {% data variables.product.prodname_unified_search %} for and that you have access to in the connected {% data variables.product.prodname_dotcom_the_website %} organization. あなたの {% data variables.product.prodname_enterprise %} 管理者と、{% data variables.product.prodname_dotcom_the_website %} 上の Organization のオーナーは、あなたのアカウントが所有しているプライベートリポジトリは検索できません。 適用可能なプライベートリポジトリを検索するには、{% data variables.product.prodname_dotcom_the_website %} および {% data variables.product.prodname_enterprise %} 上のあなたの個人アカウントに対してプライベートリポジトリ検索を有効化しなければなりません。 詳細は「[{% data variables.product.prodname_enterprise %} アカウントでのプライベートな {% data variables.product.prodname_dotcom_the_website %} リポジトリの検索を有効化する](/articles/enabling-private-github-com-repository-search-in-your-github-enterprise-server-account)」を参照してください。
+プライベートリポジトリで {% data variables.product.prodname_unified_search %} を有効にしている場合は、管理者が {% data variables.product.prodname_unified_search %} を有効にしていて、接続されている {% data variables.product.prodname_dotcom_the_website %} Organization でアクセスできるプライベートリポジトリ内しか検索できません。 あなたの {% data variables.product.prodname_enterprise %} 管理者と、{% data variables.product.prodname_dotcom_the_website %} 上の Organization のオーナーは、あなたのアカウントが所有しているプライベートリポジトリは検索できません。 適用可能なプライベートリポジトリを検索するには、{% data variables.product.prodname_dotcom_the_website %} および {% data variables.product.prodname_enterprise %} 上のあなたの個人アカウントに対してプライベートリポジトリ検索を有効化しなければなりません。 詳細は「[{% data variables.product.prodname_enterprise %} アカウントでのプライベートな {% data variables.product.prodname_dotcom_the_website %} リポジトリの検索を有効化する](/articles/enabling-private-github-com-repository-search-in-your-github-enterprise-server-account)」を参照してください。
{% endif %}
### 参考リンク
diff --git a/translations/ja-JP/content/github/searching-for-information-on-github/searching-code.md b/translations/ja-JP/content/github/searching-for-information-on-github/searching-code.md
index 12ea21485513..af17c3237102 100644
--- a/translations/ja-JP/content/github/searching-for-information-on-github/searching-code.md
+++ b/translations/ja-JP/content/github/searching-for-information-on-github/searching-code.md
@@ -11,7 +11,7 @@ versions:
github-ae: '*'
---
-{% data variables.product.product_name %} 全体にわたってグローバルにコードを検索できます。あるいは、特定のリポジトリや Organization のみのコードの検索もできます。 パブリックリポジトリのすべてにわたってコードを検索するには、{% data variables.product.product_name %} にサインインしていなければなりません。 詳細は「[GitHub 上での検索について](/articles/about-searching-on-github)」を参照してください。
+{% data reusables.search.you-can-search-globally %} 詳細については、「[GitHub での検索](/articles/about-searching-on-github)」を参照してください。
これらのコード検索の修飾子を使わなければ、コードを検索できません。 リポジトリ、ユーザまたはコミットの特定の修飾子での検索は、コードを検索する場合、うまくいきません。
@@ -21,13 +21,13 @@ versions:
コードの検索は複雑なため、検索の実行には一定の制限があります。
-- {% data reusables.search.required_login %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- {% data reusables.search.required_login %}{% endif %}
- [フォーク](/articles/about-forks)のコードは、親リポジトリより Star が多い場合に限って検索可能です。 親リポジトリより Star が少ないフォークは、コード検索ではインデックス**されません。** 親リポジトリより Star が多いフォークを検索結果に含めるためには、クエリに `fork:true` または `fork:only` を追加する必要があります。 詳細は「[フォーク内で検索する](/articles/searching-in-forks)」を参照してください。
- コード検索では、_デフォルトブランチ_のみインデックスされます。{% if currentVersion == "free-pro-team@latest" %}
- 384 KB より小さいファイルのみ検索可能です。{% else %}* 5 MB より小さいファイルのみ検索可能です。
- 各ファイルの最初の 500 KB のみ検索可能です。{% endif %}
- 500,000 より少ないファイル数のリポジトリのみ検索可能です。
-- サインインしているユーザは、すべてのパブリックリポジトリを検索可能です。
- [`filename`](#search-by-filename) の検索を除き、ソースコードを検索する場合、常に少なくとも検索単語を 1 つ含める必要があります。 たとえば[`language:javascript`](https://github.com/search?utf8=%E2%9C%93&q=language%3Ajavascript&type=Code&ref=searchresults) は有効な検索ではありませんが、[`amazing language:javascript`](https://github.com/search?utf8=%E2%9C%93&q=amazing+language%3Ajavascript&type=Code&ref=searchresults) は有効な検索です。
- 検索結果では、同一ファイルから取り出される部分は 2 つまでです。そのファイルはさらに多くの部分でヒットしている可能性があります。
- クエリの一部として次のワイルドカード文字を用いることはできません: . , : ; / \ ` ' " = * ! ? # $ & + ^ | ~ < > ( ) { } [ ]
。 検索では、これらのシンボルは単に無視されます。
diff --git a/translations/ja-JP/content/github/searching-for-information-on-github/searching-commits.md b/translations/ja-JP/content/github/searching-for-information-on-github/searching-commits.md
index 13c1fa1137e4..44ff688aae97 100644
--- a/translations/ja-JP/content/github/searching-for-information-on-github/searching-commits.md
+++ b/translations/ja-JP/content/github/searching-for-information-on-github/searching-commits.md
@@ -96,14 +96,11 @@ versions:
| org:ORGNAME
| [**test org:github**](https://github.com/search?utf8=%E2%9C%93&q=test+org%3Agithub&type=Commits) は、@github が保有するリポジトリの「test」という単語があるコミットメッセージにマッチします。 |
| repo:USERNAME/REPO
| [**language repo:defunkt/gibberish**](https://github.com/search?utf8=%E2%9C%93&q=language+repo%3Adefunkt%2Fgibberish&type=Commits) は、@defunkt の「gibberish」リポジトリにある「language」という単語があるコミットメッセージにマッチします。 |
-### パブリックリポジトリまたはプライベートリポジトリをフィルタリング
+### リポジトリの可視性によるフィルタ
-`is` 修飾子は、パブリックまたはプライベートのコミットにマッチします。
+`is` 修飾子は、指定した可視性を持つリポジトリからのコミットにマッチします。 詳細は「[リポジトリの可視性について](/github/creating-cloning-and-archiving-repositories/about-repository-visibility)」を参照してください。
-| 修飾子 | サンプル |
-| ------------ | --------------------------------------------------------------------------------------------- |
-| `is:public` | [**is:public**](https://github.com/search?q=is%3Apublic&type=Commits) は、パブリックのコミットにマッチします。 |
-| `is:private` | [**is:private**](https://github.com/search?q=is%3Aprivate&type=Commits) は、プライベートのコミットにマッチします。 |
+| 修飾子 | 例 | ------------- | ------------- |{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %} | `is:public` | [**is:public**](https://github.com/search?q=is%3Apublic&type=Commits) はパブリックリポジトリへのコミットにマッチします。{% endif %} | `is:internal` | [**is:internal**](https://github.com/search?q=is%3Ainternal&type=Commits) は内部リポジトリへのコミットにマッチします。 | `is:private` | [**is:private**](https://github.com/search?q=is%3Aprivate&type=Commits) はプライベートリポジトリへのコミットに一致します。
### 参考リンク
diff --git a/translations/ja-JP/content/github/searching-for-information-on-github/searching-for-repositories.md b/translations/ja-JP/content/github/searching-for-information-on-github/searching-for-repositories.md
index 0cf6add935df..7ac30ec6d4d7 100644
--- a/translations/ja-JP/content/github/searching-for-information-on-github/searching-for-repositories.md
+++ b/translations/ja-JP/content/github/searching-for-information-on-github/searching-for-repositories.md
@@ -10,7 +10,7 @@ versions:
github-ae: '*'
---
-{% data variables.product.product_name %} 全体にわたってグローバルにリポジトリを検索できます。あるいは、特定の Organization のみのリポジトリの検索もできます。 詳細は「[{% data variables.product.prodname_dotcom %} での検索について](/articles/about-searching-on-github)」を参照してください。
+{% data variables.product.product_location %} 全体にわたってグローバルにリポジトリを検索できます。あるいは、特定の Organization のみのリポジトリの検索もできます。 詳細は「[{% data variables.product.prodname_dotcom %} での検索について](/articles/about-searching-on-github)」を参照してください。
フォークを検索結果に含めるためには、クエリに `fork:true` または `fork:only` を追加する必要があります。 詳細は「[フォーク内で検索する](/articles/searching-in-forks)」を参照してください。
@@ -24,18 +24,18 @@ versions:
| ----------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------- |
| `in:name` | [**jquery in:name**](https://github.com/search?q=jquery+in%3Aname&type=Repositories) は、リポジトリ名に「jquery」が含まれるリポジトリにマッチします。 |
| `in:description` | [**jquery in:name,description**](https://github.com/search?q=jquery+in%3Aname%2Cdescription&type=Repositories) は、リポジトリ名または説明に「jquery」が含まれるリポジトリにマッチします。 |
-| `in:readme` | [**jquery in:readme**](https://github.com/search?q=jquery+in%3Areadme&type=Repositories) は、README ファイルで「jquery」をメンションしているリポジトリにマッチします。 |
+| `in:readme` | [**jquery in:readme**](https://github.com/search?q=jquery+in%3Areadme&type=Repositories) は、リポジトリの README ファイルで「jquery」をメンションしているリポジトリにマッチします。 |
| `repo:owner/name` | [**repo:octocat/hello-world**](https://github.com/search?q=repo%3Aoctocat%2Fhello-world) は、特定のリポジトリ名にマッチします。 |
### リポジトリの内容で検索
-`in:readme` 修飾子を使って、リポジトリの README ファイルの内容に基づいてリポジトリを検索できます。
+`in:readme` 修飾子を使用すると、リポジトリの README ファイルの内容に基づいてリポジトリを検索できます。 詳細は「[README について](/github/creating-cloning-and-archiving-repositories/about-readmes)」を参照してください。
`in:readme` は、特定の内容に基づいてリポジトリを検索する唯一の方法です。 リポジトリ内の特定のファイルや内容を検索するには、ファイルファインダー、またはコード固有の検索修飾子を使います。 詳細は「[ {% data variables.product.prodname_dotcom %}でファイルを検索する](/articles/finding-files-on-github)」および「[コードの検索](/articles/searching-code)」を参照してください。
-| 修飾子 | サンプル |
-| ----------- | ----------------------------------------------------------------------------------------------------------------------------------------- |
-| `in:readme` | [**octocat in:readme**](https://github.com/search?q=octocat+in%3Areadme&type=Repositories) は、README ファイルで「octocat」をメンションしているリポジトリにマッチします。 |
+| 修飾子 | サンプル |
+| ----------- | ------------------------------------------------------------------------------------------------------------------------------------------------ |
+| `in:readme` | [**octocat in:readme**](https://github.com/search?q=octocat+in%3Areadme&type=Repositories) は、リポジトリの README ファイルで「octocat」をメンションしているリポジトリにマッチします。 |
### ユーザまたは Organization のリポジトリ内の検索
@@ -48,7 +48,7 @@ versions:
### リポジトリのサイズで検索
-`size` 修飾子は、[不等号や範囲の修飾子](/articles/understanding-the-search-syntax)を使うことで、特定のサイズ (キロバイト) に合致するリポジトリを表示します。
+The `size` qualifier finds repositories that match a certain size (in kilobytes), using greater than, less than, and range qualifiers. 詳しい情報については、「[検索構文を理解する](/github/searching-for-information-on-github/understanding-the-search-syntax)」を参照してください。
| 修飾子 | サンプル |
| ------------------------- | ---------------------------------------------------------------------------------------------------------------------- |
@@ -59,7 +59,7 @@ versions:
### フォロワーの数の検索
-`followers` 修飾子を [不等号や範囲の修飾子](/articles/understanding-the-search-syntax)とともに使うことで、ユーザのフォロワーの数でリポジトリをフィルタリングできます。
+`followers` 修飾子と、不等号や範囲の修飾子を使用すると、リポジトリをフォローしているユーザーの数に基づいてリポジトリをフィルタリングできます。 詳しい情報については、「[検索構文を理解する](/github/searching-for-information-on-github/understanding-the-search-syntax)」を参照してください。
| 修飾子 | サンプル |
| ------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -68,7 +68,7 @@ versions:
### フォークの数で検索
-`forks` 修飾子は、[不等号や範囲の修飾子](/articles/understanding-the-search-syntax)を使って、リポジトリが持つべきフォークの数を指定します。
+`forks` 修飾子は、不等号や範囲の修飾子を使って、リポジトリが持つべきフォークの数を指定します。 詳しい情報については、「[検索構文を理解する](/github/searching-for-information-on-github/understanding-the-search-syntax)」を参照してください。
| 修飾子 | サンプル |
| ------------------------- | ------------------------------------------------------------------------------------------------------------------------- |
@@ -79,7 +79,7 @@ versions:
### Star の数で検索
-[不等号や範囲の修飾子](/articles/understanding-the-search-syntax)を使って、リポジトリの [Star](/articles/saving-repositories-with-stars) の数でリポジトリを検索できます。
+不等号や範囲の修飾子を使って、リポジトリの Star の数でリポジトリを検索できます。 詳しい情報については「[Star を付けてリポジトリを保存する](/github/getting-started-with-github/saving-repositories-with-stars)」および「[検索構文を理解する](/github/searching-for-information-on-github/understanding-the-search-syntax)」を参照してください。
| 修飾子 | サンプル |
| ------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -103,7 +103,7 @@ versions:
### 言語で検索
-リポジトリを記述した主要言語でリポジトリを検索することができます。
+リポジトリのコードの言語に基づいてリポジトリを検索できます。
| 修飾子 | サンプル |
| ------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------ |
@@ -111,7 +111,7 @@ versions:
### Topics で検索
-特定の [Topics](/articles/classifying-your-repository-with-topics) で分類されたすべてのリポジトリを見つけることができます。
+特定の Topics で分類されたすべてのリポジトリを見つけることができます。 詳細は「[トピックでリポジトリを分類する](/github/administering-a-repository/classifying-your-repository-with-topics)」を参照してください。
| 修飾子 | サンプル |
| ------------------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -119,35 +119,36 @@ versions:
### Topics の数で検索
-`topics` 修飾子を [不等号や範囲の修飾子](/articles/understanding-the-search-syntax)とともに使うことで、リポジトリに適用された [Topics ](/articles/classifying-your-repository-with-topics) の数でリポジトリを検索できます。
+`topics` 修飾子と、不等号や範囲の修飾子を使うと、リポジトリに適用された Topics の数でリポジトリを検索できます。 詳しい情報については「[Topics によるリポジトリの分類](/github/administering-a-repository/classifying-your-repository-with-topics)」および「[検索構文を理解する](/github/searching-for-information-on-github/understanding-the-search-syntax)」を参照してください。
| 修飾子 | サンプル |
| -------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------ |
| topics:n
| [**topics:5**](https://github.com/search?utf8=%E2%9C%93&q=topics%3A5&type=Repositories&ref=searchresults) は、5 つのトピックがあるリポジトリにマッチします。 |
| | [**topics:>3**](https://github.com/search?utf8=%E2%9C%93&q=topics%3A%3E3&type=Repositories&ref=searchresults) は、4 つ以上のトピックがあるリポジトリにマッチします。 |
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
### ライセンスで検索
-[ライセンス](/articles/licensing-a-repository)でリポジトリを検索できます。 特定のライセンスまたはライセンスファミリーによってリポジトリをフィルタリングするには、[ライセンスキーワード](/articles/licensing-a-repository/#searching-github-by-license-type)を使う必要があります。
+リポジトリのライセンスの種類に基づいてリポジトリを検索できます。 特定のライセンスまたはライセンスファミリーによってリポジトリをフィルタリングするには、ライセンスキーワードを使う必要があります。 詳細は「[リポジトリのライセンス](/github/creating-cloning-and-archiving-repositories/licensing-a-repository)」を参照してください。
| 修飾子 | サンプル |
| -------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| license:LICENSE_KEYWORD
| [**license:apache-2.0**](https://github.com/search?utf8=%E2%9C%93&q=license%3Aapache-2.0&type=Repositories&ref=searchresults) は、Apache ライセンス 2.0 によりライセンスされたリポジトリにマッチします。 |
-### リポジトリがパブリックかプライベートかで検索
+{% endif %}
+
+### リポジトリの可視性で検索
-リポジトリがパブリックかプライベートかどうかで検索をフィルタリングできます。
+リポジトリの可視性に基づいて検索を絞り込むことができます。 詳細は「[リポジトリの可視性について](/github/creating-cloning-and-archiving-repositories/about-repository-visibility)」を参照してください。
-| 修飾子 | サンプル |
-| ------------ | -------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `is:public` | [**is:public org:github**](https://github.com/search?q=is%3Apublic+org%3Agithub&type=Repositories&utf8=%E2%9C%93) は、パブリックな Github が保有するリポジトリにマッチします。 |
-| `is:private` | [**is:public org:github**](https://github.com/search?utf8=%E2%9C%93&q=pages+is%3Aprivate&type=Repositories) は、あなたがアクセスでき、かつ、「pages」という単語を含むプライベートリポジトリにマッチします。 |
+| 修飾子 | 例 | ------------- | ------------- |{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %} | `is:public` | [**is:public org:github**](https://github.com/search?q=is%3Apublic+org%3Agithub&type=Repositories) は、{% data variables.product.company_short %} が所有しているパブリックリポジトリにマッチします。{% endif %} | `is:internal` | [**is:internal test**](https://github.com/search?q=is%3Ainternal+test&type=Repositories) は、自分がアクセスできて「test」という単語を含む内部リポジトリにマッチします。 | `is:private` | [**is:private pages**](https://github.com/search?q=is%3Aprivate+pages&type=Repositories) は、自分がアクセスできて「pages」という単語を含むプライベートリポジトリにマッチします。
{% if currentVersion == "free-pro-team@latest" %}
### リポジトリがミラーかどうかで検索
-リポジトリがミラーかどうか、そして別の場所にホストされているかどうかでリポジトリを検索できます。 詳しい情報については、「[{% data variables.product.prodname_dotcom %} でオープンソースにコントリビュートする方法を見つける](/github/getting-started-with-github/finding-ways-to-contribute-to-open-source-on-github)」を参照してください。
+リポジトリがミラーか、それ以外にホストされているかに基づいてリポジトリを検索できます。 詳しい情報については、「[{% data variables.product.prodname_dotcom %} でオープンソースにコントリビュートする方法を見つける](/github/getting-started-with-github/finding-ways-to-contribute-to-open-source-on-github)」を参照してください。
| 修飾子 | サンプル |
| -------------- | --------------------------------------------------------------------------------------------------------------------------------------- |
@@ -158,7 +159,7 @@ versions:
### リポジトリがアーカイブされているかどうかで検索
-[アーカイブされている](/articles/about-archiving-repositories)かどうかでリポジトリを検索できます。
+アーカイブされているかどうかでリポジトリを検索できます。 詳しい情報については、「[リポジトリのアーカイブについて](/github/creating-cloning-and-archiving-repositories/about-archiving-repositories)」を参照してください。
| 修飾子 | サンプル |
| ---------------- | ---------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -166,6 +167,7 @@ versions:
| `archived:false` | [**archived:false GNOME**](https://github.com/search?utf8=%E2%9C%93&q=archived%3Afalse+GNOME&type=) は、「GNOME」という単語を含む、アーカイブされていないリポジトリにマッチします。 |
{% if currentVersion == "free-pro-team@latest" %}
+
### `good first issue` ラベルや `help wanted` ラベルの付いた Issue の数で検索
`help-wanted` ラベルや `good-first-issue` ラベルの付いた Issue の最低数があるリポジトリを、`help-wanted-issues:>n` 修飾子や `good-first-issues:>n` 修飾子によって検索できます。 詳細は、「[ラベルを使用してプロジェクトに役立つコントリビューションを促す](/github/building-a-strong-community/encouraging-helpful-contributions-to-your-project-with-labels)」を参照してください。
@@ -174,6 +176,7 @@ versions:
| -------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
| `good-first-issues:>n` | [**good-first-issues:>2 javascript**](https://github.com/search?utf8=%E2%9C%93&q=javascript+good-first-issues%3A%3E2&type=) は、「javascript」という単語を含む、`good-first-issue` ラベルが付いた3つ以上の Issue のあるリポジトリにマッチします。 |
| `help-wanted-issues:>n` | [**help-wanted-issues:>4 react**](https://github.com/search?utf8=%E2%9C%93&q=react+help-wanted-issues%3A%3E4&type=) は、「React」という単語を含む、`help-wanted` ラベルが付いた 5 つ以上の Issue のあるリポジトリにマッチします。 |
+
{% endif %}
### 参考リンク
diff --git a/translations/ja-JP/content/github/searching-for-information-on-github/searching-issues-and-pull-requests.md b/translations/ja-JP/content/github/searching-for-information-on-github/searching-issues-and-pull-requests.md
index 3cc70954c186..3bb89848681c 100644
--- a/translations/ja-JP/content/github/searching-for-information-on-github/searching-issues-and-pull-requests.md
+++ b/translations/ja-JP/content/github/searching-for-information-on-github/searching-issues-and-pull-requests.md
@@ -64,66 +64,63 @@ versions:
| `is:open` | [**performance is:open is:issue**](https://github.com/search?q=performance+is%3Aopen+is%3Aissue&type=Issues) は、「performance」という単語があるオープン Issue にマッチします。 |
| `is:closed` | [**android is:closed**](https://github.com/search?utf8=%E2%9C%93&q=android+is%3Aclosed&type=) は、「android」という単語があるクローズされた Issue とプルリクエストにマッチします。 |
-### リポジトリがパブリックかプライベートかで検索
+### リポジトリの可視性によるフィルタ
-[{% data variables.product.product_name %} のすべてにわたって検索](https://github.com/search)する場合、リポジトリがパブリックかプライベートかでフィルタリングすると便利です。 `is:public` および `is:private` を使用して、これができます。
+You can filter by the visibility of the repository containing the issues and pull requests using the `is` qualifier. 詳細は「[リポジトリの可視性について](/github/creating-cloning-and-archiving-repositories/about-repository-visibility)」を参照してください。
-| 修飾子 | サンプル |
-| ------------ | ----------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `is:public` | [**is:public**](https://github.com/search?q=is%3Apublic&type=Issues) は、すべてのパブリックなリポジトリの Issue とプルリクエストにマッチします。 |
-| `is:private` | [**is:private cupcake**](https://github.com/search?q=is%3Aprivate&type=Issues) は、あなたがアクセスできるプライベートなリポジトリにある「cupcake」という単語を含む Issue およびプルリクエストにマッチします。 |
+| Qualifier | Example | ------------- | ------------- |{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %} | `is:public` | [**is:public**](https://github.com/search?q=is%3Apublic&type=Issues) matches issues and pull requests in public repositories.{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.20" or currentVersion == "github-ae@latest" %} | `is:internal` | [**is:internal**](https://github.com/search?q=is%3Ainternal&type=Issues) matches issues and pull requests in internal repositories.{% endif %} | `is:private` | [**is:private cupcake**](https://github.com/search?q=is%3Aprivate+cupcake&type=Issues) matches issues and pull requests that contain the word "cupcake" in private repositories you can access.
### 作者で検索
`author` 修飾子によって、特定のユーザまたはインテグレーションアカウントが作成した Issue およびプルリクエストを検索できます。
-| 修飾子 | サンプル |
-| ------------------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| author:USERNAME
| [**cool author:gjtorikian**](https://github.com/search?q=cool+author%3Agjtorikian&type=Issues)は、@gjtorikian が作成した「cool」という単語がある Issue とプルリクエストにマッチします。 |
-| | [**bootstrap in:body author:mdo**](https://github.com/search?q=bootstrap+in%3Abody+author%3Amdo&type=Issues) は、本文に「bootstrap」という単語を含む @mdo が作成した Issue にマッチします。 |
-| author:app/USERNAME
| [**author:app/robot**](https://github.com/search?q=author%3Aapp%2Frobot&type=Issues) は、「robot」というインテグレーションアカウントが作成した Issue にマッチします。 |
+| 修飾子 | サンプル |
+| ------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| author:USERNAME
| [**cool author:gjtorikian**](https://github.com/search?q=cool+author%3Agjtorikian&type=Issues) matches issues and pull requests with the word "cool" that were created by @gjtorikian. |
+| | [**bootstrap in:body author:mdo**](https://github.com/search?q=bootstrap+in%3Abody+author%3Amdo&type=Issues) matches issues written by @mdo that contain the word "bootstrap" in the body. |
+| author:app/USERNAME
| [**author:app/robot**](https://github.com/search?q=author%3Aapp%2Frobot&type=Issues) matches issues created by the integration account named "robot." |
### アサインされた人で検索
-`assignee` 修飾子は、特定のユーザにアサインされた Issue およびプルリクエストを表示します。 アサインされた人がいる Issue およびプルリクエストは、_一切_検索できません。 [アサインされた人がいない Issue およびプルリクエスト](#search-by-missing-metadata)は、検索できます。
+`assignee` 修飾子は、特定のユーザにアサインされた Issue およびプルリクエストを表示します。 You cannot search for issues and pull requests that have _any_ assignee, however, you can search for [issues and pull requests that have no assignee](#search-by-missing-metadata).
-| 修飾子 | サンプル |
-| ------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| assignee:USERNAME
| [**assignee:vmg repo:libgit2/libgit2**](https://github.com/search?utf8=%E2%9C%93&q=assignee%3Avmg+repo%3Alibgit2%2Flibgit2&type=Issues) は、@vmg にアサインされた libgit2 のプロジェクト libgit2 の Issue およびプルリクエストにマッチします。 |
+| 修飾子 | サンプル |
+| ------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| assignee:USERNAME
| [**assignee:vmg repo:libgit2/libgit2**](https://github.com/search?utf8=%E2%9C%93&q=assignee%3Avmg+repo%3Alibgit2%2Flibgit2&type=Issues) matches issues and pull requests in libgit2's project libgit2 that are assigned to @vmg. |
### メンションで検索
`mentions` 修飾子は、特定のユーザーにメンションしている Issue を表示します。 詳細は「[人およびチームにメンションする](/articles/basic-writing-and-formatting-syntax/#mentioning-people-and-teams)」を参照してください。
-| 修飾子 | サンプル |
-| ------------------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------- |
-| mentions:USERNAME
| [**resque mentions:defunkt**](https://github.com/search?q=resque+mentions%3Adefunkt&type=Issues) は、@defunkt にメンションしている「resque」という単語がある Issue にマッチします。 |
+| 修飾子 | サンプル |
+| ------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| mentions:USERNAME
| [**resque mentions:defunkt**](https://github.com/search?q=resque+mentions%3Adefunkt&type=Issues) matches issues with the word "resque" that mention @defunkt. |
### Team メンションで検索
あなたが属する Organization および Team について、 `team` 修飾子により、Organization 内の一定の Team に @メンションしている Issue またはプルリクエストを表示します。 検索を行うには、これらのサンプルの名前をあなたの Organization および Team の名前に置き換えてください。
-| 修飾子 | サンプル |
-| ------------------------- | ------------------------------------------------------------------------------------ |
-| team:ORGNAME/TEAMNAME
| **team:jekyll/owners** は、`@jekyll/owners` Team がメンションされている Issue にマッチします。 |
-| | **team:myorg/ops is:open is:pr** は、`@myorg/ops` Team がメンションされているオープンなプルリクエストにマッチします。 |
+| 修飾子 | サンプル |
+| ------------------------- | ----------------------------------------------------------------------------------------------------- |
+| team:ORGNAME/TEAMNAME
| **team:jekyll/owners** matches issues where the `@jekyll/owners` team is mentioned. |
+| | **team:myorg/ops is:open is:pr** matches open pull requests where the `@myorg/ops` team is mentioned. |
### コメントした人で検索
`commenter` 修飾子は、特定のユーザからのコメントを含む Issue を検索します。
-| 修飾子 | サンプル |
-| ------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| commenter:USERNAME
| [**github commenter:defunkt org:github**](https://github.com/search?utf8=%E2%9C%93&q=github+commenter%3Adefunkt+org%3Agithub&type=Issues) は、@defunkt のコメントがあり、「github」という単語がある、GitHub が所有するリポジトリの Issue にマッチします。 |
+| 修飾子 | サンプル |
+| ------------------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| commenter:USERNAME
| [**github commenter:defunkt org:github**](https://github.com/search?utf8=%E2%9C%93&q=github+commenter%3Adefunkt+org%3Agithub&type=Issues) matches issues in repositories owned by GitHub, that contain the word "github," and have a comment by @defunkt. |
### Issue やプルリクエストに関係したユーザで検索
`involves` 修飾子は、特定のユーザが何らかの方法で関与する Issue を表示します。 `involves` 修飾子は、単一ユーザについて、`author`、`assignee`、`mentions`、および `commenter` を論理 OR でつなげます。 言い換えれば、この修飾子は、特定のユーザが作成した、当該ユーザにアサインされた、当該ユーザをメンションした、または、当該ユーザがコメントした、Issue およびプルリクエストを表示します。
-| 修飾子 | サンプル |
-| ------------------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| involves:USERNAME
| **[involves:defunkt involves:jlord](https://github.com/search?q=involves%3Adefunkt+involves%3Ajlord&type=Issues)** は、@defunkt または @jlord が関与している Issue にマッチします。 |
-| | [**NOT bootstrap in:body involves:mdo**](https://github.com/search?q=NOT+bootstrap+in%3Abody+involves%3Amdo&type=Issues)は、本文に「bootstrap」という単語を含まず、@mdo が関与している Issue にマッチします。 |
+| 修飾子 | サンプル |
+| ------------------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| involves:USERNAME
| **[involves:defunkt involves:jlord](https://github.com/search?q=involves%3Adefunkt+involves%3Ajlord&type=Issues)** matches issues either @defunkt or @jlord are involved in. |
+| | [**NOT bootstrap in:body involves:mdo**](https://github.com/search?q=NOT+bootstrap+in%3Abody+involves%3Amdo&type=Issues) matches issues @mdo is involved in that do not contain the word "bootstrap" in the body. |
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.20" or currentVersion == "github-ae@latest" %}
### リンクされた Issue とプルリクエストを検索する
diff --git a/translations/ja-JP/content/github/setting-up-and-managing-organizations-and-teams/about-your-organization-dashboard.md b/translations/ja-JP/content/github/setting-up-and-managing-organizations-and-teams/about-your-organization-dashboard.md
index b7e7c3a62a32..5257f71d4ec2 100644
--- a/translations/ja-JP/content/github/setting-up-and-managing-organizations-and-teams/about-your-organization-dashboard.md
+++ b/translations/ja-JP/content/github/setting-up-and-managing-organizations-and-teams/about-your-organization-dashboard.md
@@ -37,8 +37,8 @@ versions:
- プルリクエストのレビューコメントをサブミットする
- リポジトリをフォーク
- ウィキページを作成
- - コミットをプッシュ
- - パブリックリポジトリを作成
+ - Pushes commits.{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+ - Creates a public repository.{% endif %}
### さらなる情報
diff --git a/translations/ja-JP/content/github/setting-up-and-managing-organizations-and-teams/restricting-repository-visibility-changes-in-your-organization.md b/translations/ja-JP/content/github/setting-up-and-managing-organizations-and-teams/restricting-repository-visibility-changes-in-your-organization.md
index c639d75ff6f0..65a73ce7d5ab 100644
--- a/translations/ja-JP/content/github/setting-up-and-managing-organizations-and-teams/restricting-repository-visibility-changes-in-your-organization.md
+++ b/translations/ja-JP/content/github/setting-up-and-managing-organizations-and-teams/restricting-repository-visibility-changes-in-your-organization.md
@@ -9,7 +9,7 @@ versions:
github-ae: '*'
---
-リポジトリの可視性を変更する資格を Organization のオーナーのみに制限すること、またはプライベートからパブリックまたはパブリックからプライベートに可視性を変更することをリポジトリの管理者権限を所有するメンバーに許可することができます。
+You can restrict the ability to change repository visibility to organization owners only, or allow members with admin privileges for a repository to also change visibility.
{% warning %}
@@ -24,3 +24,7 @@ versions:
{% data reusables.organizations.member-privileges %}
5. [Repository visibility change] の下で、[**Allow members to change repository visibilities for this organization**] の選択を解除します。 
6. [**Save**] をクリックします。
+
+### 参考リンク
+
+- 「[リポジトリの可視性について](/github/creating-cloning-and-archiving-repositories/about-repository-visibility)」
diff --git a/translations/ja-JP/content/github/setting-up-and-managing-organizations-and-teams/reviewing-the-audit-log-for-your-organization.md b/translations/ja-JP/content/github/setting-up-and-managing-organizations-and-teams/reviewing-the-audit-log-for-your-organization.md
index 0192cef23867..4e355697106b 100644
--- a/translations/ja-JP/content/github/setting-up-and-managing-organizations-and-teams/reviewing-the-audit-log-for-your-organization.md
+++ b/translations/ja-JP/content/github/setting-up-and-managing-organizations-and-teams/reviewing-the-audit-log-for-your-organization.md
@@ -1,6 +1,7 @@
---
title: Organization の Audit log をレビューする
intro: 'Audit log により、Organization の管理者は Organization のメンバーによって行われたアクションをすばやくレビューできます。 これには、誰がいつ何のアクションを実行したかなどの詳細が残されます。'
+miniTocMaxHeadingLevel: 4
redirect_from:
- /articles/reviewing-the-audit-log-for-your-organization
versions:
@@ -11,7 +12,7 @@ versions:
### Audit log にアクセスする
-Audit log には、過去 90 日間に行われた行動が一覧表示されます。 Organization の Audit log にアクセスできるのはオーナーのみです。
+The audit log lists events triggered by activities that affect your organization within the last 90 days. Organization の Audit log にアクセスできるのはオーナーのみです。
{% data reusables.profile.access_profile %}
{% data reusables.profile.access_org %}
@@ -26,73 +27,111 @@ Audit log には、過去 90 日間に行われた行動が一覧表示されま
特定のイベントを検索するには、クエリで `action` 修飾子を使用します。 Audit log に一覧表示されるアクションは以下のカテゴリに分類されます。
-| カテゴリー名 | 説明 |
-| ------------------------------------------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |{% if currentVersion == "free-pro-team@latest" %}
-| `アカウント` | Organization アカウントに関連するすべてのアクティビティが対象です。{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-| `支払い` | Organization の支払いに関連するすべてのアクティビティが対象です。{% endif %}
-| `discussion_post` | Team ページに投稿されたディスカッションに関連するすべてのアクティビティが対象です。 |
-| `discussion_post_reply` | Team ページに投稿されたディスカッションへの返答に関連するすべてのアクティビティが対象です。 |
-| `フック` | webhookに関連するすべてのアクティビティを含みます。 |
-| `integration_installation_request` | Organization 内で使用するインテグレーションをオーナーが承認するよう求める、 Organization メンバーからのリクエストに関連するすべてのアクティビティが対象です。 |{% if currentVersion == "free-pro-team@latest" %}
-| `marketplace_agreement_signature` | {% data variables.product.prodname_marketplace %} Developer Agreement の署名に関連するすべての活動が対象です。 |
-| `marketplace_listing` | Contains all activities related to listing apps in {% data variables.product.prodname_marketplace %}.{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
-| `members_can_create_pages` | Organization のリポジトリについて {% data variables.product.prodname_pages %} サイトの公開を無効化することに関連するすべてのアクティビティが対象です。 詳細については、「[Organization について {% data variables.product.prodname_pages %} サイトの公開を制限する](/github/setting-up-and-managing-organizations-and-teams/disabling-publication-of-github-pages-sites-for-your-organization)」を参照してください。 |{% endif %}
-| `org` | Organization メンバーシップに関連するすべてのアクティビティが対象です。{% if currentVersion == "free-pro-team@latest" %}
-| `org_credential_authorization` | Contains all activities related to authorizing credentials for use with SAML single sign-on.{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "github-ae@latest" %}
-| `organization_label` | Organization のリポジトリのデフォルトラベルに関連するすべてのアクティビティが対象です。{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-| `payment_method` | Organization の GitHub への支払い方法に関連するすべてのアクティビティが対象です。{% endif %}
-| `profile_picture` | Organization のプロフィール画像に関連するすべてのアクティビティが対象です。 |
-| `project` | プロジェクト ボードに関連するすべての活動が対象です。 |
-| `protected_branch` | 保護されたブランチ関連するすべてのアクティビティが対象です。 |
-| `repo` | Organization によって所有されていリポジトリに関連するすべてのアクティビティが対象です。{% if currentVersion == "free-pro-team@latest" %}
-| `repository_content_analysis` | [プライベート リポジトリに対するデータの使用を有効または無効にする](/articles/about-github-s-use-of-your-data)に関連するすべての活動が対象です。 |
-| `repository_dependency_graph` | Contains all activities related to [enabling or disabling the dependency graph for a private repository](/github/visualizing-repository-data-with-graphs/exploring-the-dependencies-and-dependents-of-a-repository).{% endif %}{% if currentVersion != "github-ae@latest" %}
-| `repository_vulnerability_alert` | Contains all activities related to [{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot %}{% else %}security{% endif %} alerts for vulnerable dependencies](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies).{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-| `sponsors` | Contains all events related to sponsor buttons (see "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)"){% endif %}{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-| `Team` | Organization の Team に関連するすべてのアクティビティが対処です。{% endif %}
-| `team_discussions` | Organization の Team ディスカッションに関連するすべてのアクティビティが対象です。 |
+| カテゴリ名 | 説明 |
+| ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |{% if currentVersion == "free-pro-team@latest" %}
+| [`アカウント`](#account-category-actions) | Contains all activities related to your organization account. |
+| [`advisory_credit`](#advisory_credit-category-actions) | Contains all activities related to crediting a contributor for a security advisory in the {% data variables.product.prodname_advisory_database %}. For more information, see "[About {% data variables.product.prodname_dotcom %} Security Advisories](/github/managing-security-vulnerabilities/about-github-security-advisories)." |
+| [`支払い`](#billing-category-actions) | Contains all activities related to your organization's billing. |
+| [`dependabot_alerts`](#dependabot_alerts-category-actions) | Contains organization-level configuration activities for {% data variables.product.prodname_dependabot %} alerts in existing repositories. 詳しい情報については、「[脆弱性のある依存関係に対するアラートについて](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies)」を参照してください。 |
+| [`dependabot_alerts_new_repos`](#dependabot_alerts_new_repos-category-actions) | Contains organization-level configuration activities for {% data variables.product.prodname_dependabot %} alerts in new repositories created in the organization. |
+| [`dependabot_security_updates`](#dependabot_security_updates-category-actions) | Contains organization-level configuration activities for {% data variables.product.prodname_dependabot_security_updates %} in existing repositories. 詳しい情報については、「[{% data variables.product.prodname_dependabot_security_updates %} を設定する](/github/managing-security-vulnerabilities/configuring-dependabot-security-updates)」を参照してください。 |
+| [`dependabot_security_updates_new_repos`](#dependabot_security_updates_new_repos-category-actions) | Contains organization-level configuration activities for {% data variables.product.prodname_dependabot_security_updates %} for new repositories created in the organization. |
+| [`dependency_graph`](#dependency_graph-category-actions) | Contains organization-level configuration activities for dependency graphs for repositories. 詳しい情報については、「[依存関係グラフについて](/github/visualizing-repository-data-with-graphs/about-the-dependency-graph)」を参照してください。 |
+| [`dependency_graph_new_repos`](#dependency_graph_new_repos-category-actions) | Contains organization-level configuration activities for new repositories created in the organization.{% endif %}
+| [`discussion_post`](#discussion_post-category-actions) | Team ページに投稿されたディスカッションに関連するすべてのアクティビティが対象です。 |
+| [`discussion_post_reply`](#discussion_post_reply-category-actions) | Team ページに投稿されたディスカッションへの返答に関連するすべてのアクティビティが対象です。 |
+| [`フック`](#hook-category-actions) | webhookに関連するすべてのアクティビティを含みます。 |
+| [`integration_installation_request`](#integration_installation_request-category-actions) | Organization 内で使用するインテグレーションをオーナーが承認するよう求める、 Organization メンバーからのリクエストに関連するすべてのアクティビティが対象です。 |
+| [`Issue`](#issue-category-actions) | Contains activities related to deleting an issue. |{% if currentVersion == "free-pro-team@latest" %}
+| [`marketplace_agreement_signature`](#marketplace_agreement_signature-category-actions) | {% data variables.product.prodname_marketplace %} Developer Agreement の署名に関連するすべての活動が対象です。 |
+| [`marketplace_listing`](#marketplace_listing-category-actions) | Contains all activities related to listing apps in {% data variables.product.prodname_marketplace %}.{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
+| [`members_can_create_pages`](#members_can_create_pages-category-actions) | Organization のリポジトリについて {% data variables.product.prodname_pages %} サイトの公開を無効化することに関連するすべてのアクティビティが対象です。 詳細については、「[Organization について {% data variables.product.prodname_pages %} サイトの公開を制限する](/github/setting-up-and-managing-organizations-and-teams/disabling-publication-of-github-pages-sites-for-your-organization)」を参照してください。 |{% endif %}
+| [`org`](#org-category-actions) | Contains activities related to organization membership.{% if currentVersion == "free-pro-team@latest" %}
+| [`org_credential_authorization`](#org_credential_authorization-category-actions) | Contains all activities related to authorizing credentials for use with SAML single sign-on.{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "github-ae@latest" %}
+| [`organization_label`](#organization_label-category-actions) | Contains all activities related to default labels for repositories in your organization.{% endif %}
+| [`oauth_application`](#oauth_application-category-actions) | Contains all activities related to OAuth Apps. |{% if currentVersion == "free-pro-team@latest" %}
+| [`payment_method`](#payment_method-category-actions) | Organization の GitHub への支払い方法に関連するすべてのアクティビティが対象です。{% endif %}
+| [`profile_picture`](#profile_picture-category-actions) | Organization のプロフィール画像に関連するすべてのアクティビティが対象です。 |
+| [`project`](#project-category-actions) | プロジェクト ボードに関連するすべての活動が対象です。 |
+| [`protected_branch`](#protected_branch-category-actions) | 保護されたブランチ関連するすべてのアクティビティが対象です。 |
+| [`repo`](#repo-category-actions) | Contains activities related to the repositories owned by your organization.{% if currentVersion == "free-pro-team@latest" %}
+| [`repository_advisory`](#repository_advisory-category-actions) | Contains repository-level activities related to security advisories in the {% data variables.product.prodname_advisory_database %}. For more information, see "[About {% data variables.product.prodname_dotcom %} Security Advisories](/github/managing-security-vulnerabilities/about-github-security-advisories)." |
+| [`repository_content_analysis`](#repository_content_analysis-category-actions) | Contains all activities related to [enabling or disabling data use for a private repository](/articles/about-github-s-use-of-your-data).{% endif %}{% if currentVersion != "github-ae@latest" %}
+| [`repository_dependency_graph`](#repository_dependency_graph-category-actions) | Contains repository-level activities related to enabling or disabling the dependency graph for a |
+| {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repository. For more information, see "[About the dependency graph](/github/visualizing-repository-data-with-graphs/about-the-dependency-graph)."{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %} | |
+| [`repository_secret_scanning`](#repository_secret_scanning-category-actions) | Contains repository-level activities related to secret scanning. 詳しい情報については、「[シークレットスキャニングについて](/github/administering-a-repository/about-secret-scanning)」を参照してください。 |{% endif %}{% if currentVersion != "github-ae@latest" %}
+| [`repository_vulnerability_alert`](#repository_vulnerability_alert-category-actions) | Contains all activities related to [{% data variables.product.prodname_dependabot_alerts %} for vulnerable dependencies](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies).{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| [`repository_vulnerability_alerts`](#repository_vulnerability_alerts-category-actions) | Contains repository-level configuration activities for {% data variables.product.prodname_dependabot %} alerts. |{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %}
+| [`secret_scanning`](#secret_scanning-category-actions) | Contains organization-level configuration activities for secret scanning in existing repositories. 詳しい情報については、「[シークレットスキャニングについて](/github/administering-a-repository/about-secret-scanning)」を参照してください。 |
+| [`secret_scanning_new_repos`](#secret_scanning_new_repos-category-actions) | Contains organization-level configuration activities for secret scanning for new repositories created in the organization. |{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| [`sponsors`](#sponsors-category-actions) | Contains all events related to sponsor buttons (see "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)"){% endif %}{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
+| [`Team`](#team-category-actions) | Organization の Team に関連するすべてのアクティビティが対処です。{% endif %}
+| [`team_discussions`](#team_discussions-category-actions) | Organization の Team ディスカッションに関連するすべてのアクティビティが対象です。 |
次の用語を使用すれば、特定の一連の行動を検索できます。 例:
* `action:team`はteamカテゴリ内でグループ化されたすべてのイベントを検索します。
* `-action:hook` は webhook カテゴリ内のすべてのイベントを除外します。
-各カテゴリには、フィルタリングできる一連の関連イベントがあります。 例:
+Each category has a set of associated actions that you can filter on. 例:
* `action:team.create`はTeamが作成されたすべてのイベントを検索します。
* `-action:hook.events_changed` は webhook の変更されたすべてのイベントを除外します。
-以下リストで、使用できるカテゴリと関連するイベントを説明します。
-
-{% if currentVersion == "free-pro-team@latest" %}- [`account` カテゴリ](#the-account-category)
-- [`billing` カテゴリ](#the-billing-category){% endif %}
-- [`discussion_post` カテゴリ](#the-discussion_post-category)
-- [`discussion_post_reply` カテゴリ](#the-discussion_post_reply-category)
-- [`hook` カテゴリ](#the-hook-category)
-- [`integration_installation_request` カテゴリ](#the-integration_installation_request-category)
-- [`issue` カテゴリ](#the-issue-category){% if currentVersion == "free-pro-team@latest" %}
-- [`marketplace_agreement_signature` カテゴリ](#the-marketplace_agreement_signature-category)
-- [The `marketplace_listing` category](#the-marketplace_listing-category){% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
-- [`members_can_create_pages` カテゴリ](#the-members_can_create_pages-category){% endif %}
-- [`org` カテゴリ](#the-org-category){% if currentVersion == "free-pro-team@latest" %}
-- [The `org_credential_authorization` category](#the-org_credential_authorization-category){% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "github-ae@latest" %}
-- [`organization_label` カテゴリ](#the-organization_label-category){% endif %}
-- [`oauth_application` カテゴリ](#the-oauth_application-category){% if currentVersion == "free-pro-team@latest" %}
-- [`payment_method` カテゴリ](#the-payment_method-category){% endif %}
-- [`profile_picture` カテゴリ](#the-profile_picture-category)
-- [`project` カテゴリ](#the-project-category)
-- [`protected_branch` カテゴリ](#the-protected_branch-category)
-- [`repo` カテゴリ](#the-repo-category){% if currentVersion == "free-pro-team@latest" %}
-- [`repository_content_analysis` カテゴリ](#the-repository_content_analysis-category)
-- [The `repository_dependency_graph` category](#the-repository_dependency_graph-category){% endif %}{% if currentVersion != "github-ae@latest" %}
-- [The `repository_vulnerability_alert` category](#the-repository_vulnerability_alert-category){% endif %}{% if currentVersion == "free-pro-team@latest" %}
-- [The `sponsors` category](#the-sponsors-category){% endif %}{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-- [`team` カテゴリ](#the-team-category){% endif %}
-- [`team_discussions` カテゴリ](#the-team_discussions-category)
+#### アクション時間に基づく検索
+
+Use the `created` qualifier to filter events in the audit log based on when they occurred. {% data reusables.time_date.date_format %} {% data reusables.time_date.time_format %}
+
+{% data reusables.search.date_gt_lt %} 例:
+
+ * `created:2014-07-08` は、2014 年 7 月 8 日に発生したイベントをすべて検索します。
+ * `created:>=2014-07-08` は、2014 年 7 月 8 日かそれ以降に生じたすべてのイベントを検索します。
+ * `created:<=2014-07-08`は、2014 年 7 月 8 日かそれ以前に生じたすべてのイベントを検索します。
+ * `created:2014-07-01..2014-07-31`は、2014 年 7 月に起きたすべてのイベントを検索します。
+
+Audit log には過去 90 日間のデータが存在しますが、`created` 修飾子を使用すればそれより前のイベントを検索できます。
+
+#### 場所に基づく検索
+
+Using the qualifier `country`, you can filter events in the audit log based on the originating country. 国の 2 文字のショートコードまたはフル ネームを使用できます。 名前に空白がある国は引用符で囲む必要があることに注意してください。 例:
+
+ * `country:de` は、ドイツで発生したイベントをすべて検索します。
+ * `country:Mexico` はメキシコで発生したすべてのイベントを検索します。
+ * `country:"United States"` はアメリカ合衆国で発生したすべてのイベントを検索します。
+
+{% if currentVersion == "free-pro-team@latest" %}
+### Audit log をエクスポートする
+
+{% data reusables.audit_log.export-log %}
+{% data reusables.audit_log.exported-log-keys-and-values %}
+{% endif %}
+
+### Audit log API を使用する
+
+{% note %}
+
+**メモ**: Audit log API は、{% data variables.product.prodname_enterprise %} を使用している Organization が利用できます。 {% data reusables.gated-features.more-info-org-products %}
+
+{% endnote %}
+
+IP の安全性を保ち、Organization のコンプライアンスを守るため、Audit log API を使って、Audit log データのコピーを保存し、モニタリングできます。
+* Organization またはリポジトリの設定へのアクセス
+* 権限の変更
+* Organization、リポジトリ、または Team におけるユーザの追加や削除
+* 管理者に昇格しているユーザ
+* GitHub App の権限の変更
+
+GraphQL のレスポンスには、90 日から 120 日までのデータを含めることができます。
+
+たとえば、GraphQL にリクエストして、Organization に新しく追加された Organization メンバー全員を表示できます。 詳細は「[GraphQL API Audit Log](/graphql/reference/interfaces#auditentry/)」を参照してください。
+
+### Audit log actions
+
+An overview of some of the most common actions that are recorded as events in the audit log.
{% if currentVersion == "free-pro-team@latest" %}
-##### `account` カテゴリ
+#### `account` category actions
| アクション | 説明 |
| ----------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------ |
@@ -101,30 +140,81 @@ Audit log には、過去 90 日間に行われた行動が一覧表示されま
| `pending_plan_change` | Organization のオーナーまたは支払いマネージャーが[支払い済みサブスクリプションをキャンセルまたはダウングレードする](/articles/how-does-upgrading-or-downgrading-affect-the-billing-process/)ときにトリガーされます。 |
| `pending_subscription_change` | [{% data variables.product.prodname_marketplace %} の無料トライアルが始まるか期限切れになる](/articles/about-billing-for-github-marketplace/)ときにトリガーされます。 |
-##### `billing` カテゴリ
+#### `advisory_credit` category actions
+
+| アクション | 説明 |
+| --------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `accept` | Triggered when someone accepts credit for a security advisory. 詳しい情報については、「[セキュリティアドバイザリを編集する](/github/managing-security-vulnerabilities/editing-a-security-advisory)」を参照してください。 |
+| `create` | Triggered when the administrator of a security advisory adds someone to the credit section. |
+| `decline` | Triggered when someone declines credit for a security advisory. |
+| `destroy` | Triggered when the administrator of a security advisory removes someone from the credit section. |
+
+#### `billing` category actions
| アクション | 説明 |
| --------------------- | ---------------------------------------------------------------------------------------------------------------------------------- |
| `change_billing_type` | Organization が[{% data variables.product.prodname_dotcom %} の支払い方法を変更する](/articles/adding-or-editing-a-payment-method)ときにトリガーされます。 |
| `change_email` | Organization の[支払い請求先メール アドレス](/articles/setting-your-billing-email)が変わるときにトリガーされます。 |
+#### `dependabot_alerts` category actions
+
+| アクション | 説明 |
+| --------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| `disable` | Triggered when an organization owner disables {% data variables.product.prodname_dependabot_alerts %} for all existing {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repositories. For more information, see "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)." |
+| `enable` | Triggered when an organization owner enables {% data variables.product.prodname_dependabot_alerts %} for all existing {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repositories. |
+
+#### `dependabot_alerts_new_repos` category actions
+
+| アクション | 説明 |
+| --------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `disable` | Triggered when an organization owner disables {% data variables.product.prodname_dependabot_alerts %} for all new {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repositories. For more information, see "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)." |
+| `enable` | Triggered when an organization owner enbles {% data variables.product.prodname_dependabot_alerts %} for all new {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repositories. |
+
+#### `dependabot_security_updates` category actions
+
+| アクション | 説明 |
+| --------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `disable` | Triggered when an organization owner disables {% data variables.product.prodname_dependabot_security_updates %} for all existing repositories. For more information, see "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)." |
+| `enable` | Triggered when an organization owner enables {% data variables.product.prodname_dependabot_security_updates %} for all existing repositories. |
+
+#### `dependabot_security_updates_new_repos` category actions
+
+| アクション | 説明 |
+| --------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `disable` | Triggered when an organization owner disables {% data variables.product.prodname_dependabot_security_updates %} for all new repositories. For more information, see "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)." |
+| `enable` | Triggered when an organization owner enables {% data variables.product.prodname_dependabot_security_updates %} for all new repositories. |
+
+#### `dependency_graph` category actions
+
+| アクション | 説明 |
+| --------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `disable` | Triggered when an organization owner disables the dependency graph for all existing repositories. For more information, see "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)." |
+| `enable` | Triggered when an organization owner enables the dependency graph for all existing repositories. |
+
+#### `dependency_graph_new_repos` category actions
+
+| アクション | 説明 |
+| --------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `disable` | Triggered when an organization owner disables the dependency graph for all new repositories. For more information, see "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)." |
+| `enable` | Triggered when an organization owner enables the dependency graph for all new repositories. |
+
{% endif %}
-##### `discussion_post` カテゴリ
+#### `discussion_post` category actions
| アクション | 説明 |
| --------- | ------------------------------------------------------------------------------------------------ |
| `update` | [Team ディスカッションの投稿が編集される](/articles/managing-disruptive-comments/#editing-a-comment)ときにトリガーされます。 |
| `destroy` | [Team ディスカッションの投稿が削除される](/articles/managing-disruptive-comments/#deleting-a-comment)ときにトリガーされます。 |
-##### `discussion_post_reply` カテゴリ
+#### `discussion_post_reply` category actions
| アクション | 説明 |
| --------- | ---------------------------------------------------------------------------------------------------- |
| `update` | [Team ディスカッションの投稿への返答が編集される](/articles/managing-disruptive-comments/#editing-a-comment)ときにトリガーされます。 |
| `destroy` | [Team ディスカッションの投稿への返答が削除される](/articles/managing-disruptive-comments/#deleting-a-comment)ときにトリガーされます。 |
-##### `hook` カテゴリ
+#### `hook` category actions
| アクション | 説明 |
| ---------------- | ------------------------------------------------------------------------------- |
@@ -133,14 +223,14 @@ Audit log には、過去 90 日間に行われた行動が一覧表示されま
| `destroy` | 既存のフックがリポジトリから削除されたときにトリガーされます。 |
| `events_changed` | フックでのイベントが変更された場合にトリガーされます。 |
-##### `integration_installation_request` カテゴリ
+#### `integration_installation_request` category actions
| アクション | 説明 |
| -------- | ---------------------------------------------------------------------------------------------------------------------------------- |
| `create` | Organization のメンバーが、Organization 内で使用するために、Organization のオーナーにインテグレーションをインストールすることを要求するときにトリガーされます。 |
| `close` | Organization 内で使用するためにインテグレーションをインストールする要求が、Organization のオーナーにより承認または拒否されるか、あるいは要求を公開した Organization のメンバーによりキャンセルされるときにトリガーされます。 |
-##### `issue` カテゴリ
+#### `issue` category actions
| アクション | 説明 |
| --------- | -------------------------------------------------------------------------------------- |
@@ -148,13 +238,13 @@ Audit log には、過去 90 日間に行われた行動が一覧表示されま
{% if currentVersion == "free-pro-team@latest" %}
-##### `marketplace_agreement_signature` カテゴリ
+#### `marketplace_agreement_signature` category actions
| アクション | 説明 |
| -------- | --------------------------------------------------------------------------------------- |
| `create` | {% data variables.product.prodname_marketplace %} Developer Agreement に署名するときにトリガーされます。 |
-##### `marketplace_listing` カテゴリ
+#### `marketplace_listing` category actions
| アクション | 説明 |
| --------- | ----------------------------------------------------------------------------------- |
@@ -168,7 +258,7 @@ Audit log には、過去 90 日間に行われた行動が一覧表示されま
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
-##### `members_can_create_pages` カテゴリ
+#### `members_can_create_pages` category actions
詳細については、「[Organization について {% data variables.product.prodname_pages %} サイトの公開を制限する](/github/setting-up-and-managing-organizations-and-teams/disabling-publication-of-github-pages-sites-for-your-organization)」を参照してください。
@@ -179,7 +269,7 @@ Audit log には、過去 90 日間に行われた行動が一覧表示されま
{% endif %}
-##### `org` カテゴリ
+#### `org` category actions
| アクション | 説明 |
| -------------------------------------------------------------------------------------------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |{% if currentVersion == "free-pro-team@latest"%}
@@ -222,7 +312,7 @@ Audit log には、過去 90 日間に行われた行動が一覧表示されま
| `update_terms_of_service` | Organization が標準利用規約と企業向け利用規約を切り替えるときにトリガーされます。 詳細は「[企業利用規約にアップグレードする](/articles/upgrading-to-the-corporate-terms-of-service)」を参照してください。{% endif %}
{% if currentVersion == "free-pro-team@latest" %}
-##### `org_credential_authorization` カテゴリ
+#### `org_credential_authorization` category actions
| アクション | 説明 |
| -------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -233,7 +323,7 @@ Audit log には、過去 90 日間に行われた行動が一覧表示されま
{% endif %}
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "github-ae@latest" %}
-##### `organization_label` カテゴリ
+#### `organization_label` category actions
| アクション | 説明 |
| --------- | -------------------------- |
@@ -243,7 +333,7 @@ Audit log には、過去 90 日間に行われた行動が一覧表示されま
{% endif %}
-##### `oauth_application` カテゴリ
+#### `oauth_application` category actions
| アクション | 説明 |
| --------------- | ------------------------------------------------------------------------------------------ |
@@ -255,7 +345,7 @@ Audit log には、過去 90 日間に行われた行動が一覧表示されま
{% if currentVersion == "free-pro-team@latest" %}
-##### `payment_method` カテゴリ
+#### `payment_method` category actions
| アクション | 説明 |
| -------- | -------------------------------------------------------------------- |
@@ -265,12 +355,12 @@ Audit log には、過去 90 日間に行われた行動が一覧表示されま
{% endif %}
-##### `profile_picture` カテゴリ
+#### `profile_picture` category actions
| アクション | 説明 |
| ------ | -------------------------------------------- |
| update | Organization のプロファイル写真を設定または更新するときにトリガーされます。 |
-##### `project` カテゴリ
+#### `project` category actions
| アクション | 説明 |
| ------------------------ | -------------------------------------------------------------------------------------- |
@@ -284,7 +374,7 @@ Audit log には、過去 90 日間に行われた行動が一覧表示されま
| `update_team_permission` | Team のプロジェクト ボードの権限レベルが変更されるとき、または Team がプロジェクト ボードに追加または削除されるときにトリガーされます。 |
| `update_user_permission` | Organization のメンバーまたは外部コラボレーターがプロジェクト ボードに追加または削除されるとき、または彼らの権限レベルが変更されている場合にトリガーされます。 |
-##### `protected_branch` カテゴリ
+#### `protected_branch` category actions
| アクション | 説明 |
| ----------------------------------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -304,14 +394,14 @@ Audit log には、過去 90 日間に行われた行動が一覧表示されま
| `update_linear_history_requirement_enforcement_level` | 保護されたブランチについて、必須の直線状のコミット履歴が有効化または無効化されるときにトリガーされます。 |
{% endif %}
-##### `repo` カテゴリ
+#### `repo` category actions
| アクション | 説明 |
| ------------------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `access` | Organization が所有するリポジトリが["プライベート" から "パブリック" に切り替えられる](/articles/making-a-private-repository-public) (またはその逆) ときにトリガーされます。 |
+| `access` | Triggered when a user [changes the visibility](/github/administering-a-repository/setting-repository-visibility) of a repository in the organization. |
| `add_member` | ユーザーが[リポジトリへのコラボレーションアクセスへの招待](/articles/inviting-collaborators-to-a-personal-repository)を受諾するときにトリガーされます。 |
| `add_topic` | リポジトリの管理者がリポジトリに[トピックを追加する](/articles/classifying-your-repository-with-topics)ときにトリガーされます。 |
-| `archived` | Triggered when a repository admin [archives a repository](/articles/about-archiving-repositories).{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
+| `archived` | リポジトリの管理者が[リポジトリをアーカイブする](/articles/about-archiving-repositories)ときにトリガーされます。{% if enterpriseServerVersions contains currentVersion %}
| `config.disable_anonymous_git_access` | 公開リポジトリで[匿名の Git 読み取りアクセスが無効になる](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)ときにトリガーされます。 |
| `config.enable_anonymous_git_access` | 公開リポジトリで[匿名の Git 読み取りアクセスが有効になる](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)ときにトリガーされます。 |
| `config.lock_anonymous_git_access` | リポジトリの[匿名の Git 読み取りアクセス設定がロックされる](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)ときにトリガーされます。 |
@@ -334,35 +424,80 @@ Audit log には、過去 90 日間に行われた行動が一覧表示されま
{% if currentVersion == "free-pro-team@latest" %}
-##### `repository_content_analysis` カテゴリ
+#### `repository_advisory` category actions
+
+| アクション | 説明 |
+| ------------------ | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| `close` | Triggered when someone closes a security advisory. For more information, see "[About {% data variables.product.prodname_dotcom %} Security Advisories](/github/managing-security-vulnerabilities/about-github-security-advisories)." |
+| `cve_request` | Triggered when someone requests a CVE (Common Vulnerabilities and Exposures) number from {% data.variables.product.prodname_dotcom %} for a draft security advisory. |
+| `github_broadcast` | Triggered when {% data.variables.product.prodname_dotcom %} makes a security advisory public in the {% data variables.product.prodname_advisory_database %}. |
+| `github_withdraw` | Triggered when {% data.variables.product.prodname_dotcom %} withdraws a security advisory that was published in error. |
+| `オープン` | Triggered when someone opens a draft security advisory. |
+| `publish` | Triggered when someone publishes a security advisory. |
+| `さいお` | Triggered when someone reopens as draft security advisory. |
+| `update` | Triggered when someone edits a draft or published security advisory. |
+
+#### `repository_content_analysis` category actions
| アクション | 説明 |
| --------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| `enable` | Organization のオーナーまたはリポジトリへの管理者アクセス権を所有する人が[プライベート リポジトリに対してデータ使用設定を有効にする](/github/understanding-how-github-uses-and-protects-your-data/managing-data-use-settings-for-your-private-repository)ときにトリガーされます。 |
| `disable` | Organization のオーナーまたはリポジトリへの管理者アクセス権を所有する人が[プライベート リポジトリに対してデータ使用設定を無効にする](/github/understanding-how-github-uses-and-protects-your-data/managing-data-use-settings-for-your-private-repository)ときにトリガーされます。 |
-##### `repository_dependency_graph` カテゴリ
+{% endif %}{% if currentVersion != "github-ae@latest" %}
-| アクション | 説明 |
-| --------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `enable` | リポジトリのオーナーまたはリポジトリへの管理者アクセス権を所有する人が[プライベート リポジトリに対して依存グラフを有効にする](/github/visualizing-repository-data-with-graphs/exploring-the-dependencies-and-dependents-of-a-repository)ときにトリガーされます。 |
-| `disable` | リポジトリのオーナーまたはリポジトリへの管理者アクセス権を所有する人が[プライベート リポジトリに対して依存グラフを無効にする](/github/visualizing-repository-data-with-graphs/exploring-the-dependencies-and-dependents-of-a-repository)ときにトリガーされます。 |
+#### `repository_dependency_graph` category actions
+
+| アクション | 説明 |
+| --------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `disable` | Triggered when a repository owner or person with admin access to the repository disables the dependency graph for a {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repository. 詳しい情報については、「[依存関係グラフについて](/github/visualizing-repository-data-with-graphs/about-the-dependency-graph)」を参照してください。 |
+| `enable` | Triggered when a repository owner or person with admin access to the repository enables the dependency graph for a {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repository. |
+
+{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %}
+#### `repository_secret_scanning` category actions
+
+| アクション | 説明 |
+| --------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `disable` | Triggered when a repository owner or person with admin access to the repository disables secret scanning for a {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repository. 詳しい情報については、「[シークレットスキャニングについて](/github/administering-a-repository/about-secret-scanning)」を参照してください。 |
+| `enable` | Triggered when a repository owner or person with admin access to the repository enables secret scanning for a {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repository. |
+
+{% endif %}{% if currentVersion != "github-ae@latest" %}
+#### `repository_vulnerability_alert` category actions
+
+| アクション | 説明 |
+| -------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `create` | Triggered when {% data variables.product.product_name %} creates a {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot %}{% else %}security{% endif %} alert for a repository that uses a vulnerable dependency. 詳しい情報については、「[脆弱性のある依存関係に対するアラートについて](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies)」を参照してください。 |
+| `却下` | Triggered when an organization owner or person with admin access to the repository dismisses a {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot %}{% else %}security{% endif %} alert about a vulnerable dependency. |
+| `解決` | Triggered when someone with write access to a repository pushes changes to update and resolve a vulnerability in a project dependency. |
+
+{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+#### `repository_vulnerability_alerts` category actions
+
+| アクション | 説明 |
+| ------------------------ | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| `authorized_users_teams` | Triggered when an organization owner or a person with admin permissions to the repository updates the list of people or teams authorized to receive {% data variables.product.prodname_dependabot_alerts %} for vulnerable dependencies in the repository. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-security-alerts)." |
+| `disable` | Triggered when a repository owner or person with admin access to the repository disables {% data variables.product.prodname_dependabot_alerts %}. |
+| `enable` | Triggered when a repository owner or person with admin access to the repository enables {% data variables.product.prodname_dependabot_alerts %}. |
+
+{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %}
+#### `secret_scanning` category actions
+
+| アクション | 説明 |
+| --------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `disable` | Triggered when an organization owner disables secret scanning for all existing{% if currentVersion == "free-pro-team@latest" %}, private{% endif %} repositories. 詳しい情報については、「[シークレットスキャニングについて](/github/administering-a-repository/about-secret-scanning)」を参照してください。 |
+| `enable` | Triggered when an organization owner enables secret scanning for all existing{% if currentVersion == "free-pro-team@latest" %}, private{% endif %} repositories. |
+
+#### `secret_scanning_new_repos` category actions
+
+| アクション | 説明 |
+| --------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `disable` | Triggered when an organization owner disables secret scanning for all new {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repositories. 詳しい情報については、「[シークレットスキャニングについて](/github/administering-a-repository/about-secret-scanning)」を参照してください。 |
+| `enable` | Triggered when an organization owner enables secret scanning for all new {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repositories. |
-{% endif %}
-{% if currentVersion != "github-ae@latest" %}
-##### `repository_vulnerability_alert` カテゴリ
-
-| アクション | 説明 |
-| ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `create` | {% data variables.product.product_name %} が特定のリポジトリで[脆弱な依存性に対する{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot %}{% else %}セキュリティ{% endif %}アラート](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies)を作成するときにトリガーされます。 |
-| `解決` | リポジトリへの書き込みアクセス権を所有する人が、プロジェクトの依存関係で、[脆弱性を更新して解決するために変更をプッシュする](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies)ときにトリガーされます。 |
-| `却下` | Organization のオーナーまたはリポジトリへの管理者アクセス権を所有する人は |
-| 脆弱な依存関係についての {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot %}{% else %}セキュリティ{% endif %}アラートを却下するときにトリガーされます。{% if currentVersion == "free-pro-team@latest" %} | |
-| `authorized_users_teams` | Triggered when an organization owner or a member with admin permissions to the repository [updates the list of people or teams authorized to receive {% data variables.product.prodname_dependabot_alerts %}](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-dependabot-alerts) for vulnerable dependencies in the repository.{% endif %}
{% endif %}
{% if currentVersion == "free-pro-team@latest" %}
-##### `sponsors` カテゴリ
+#### `sponsors` category actions
| アクション | 説明 |
| ----------------------------------- | ------------------------------------------------------------------------------------------------------------------------------- |
@@ -371,7 +506,7 @@ Audit log には、過去 90 日間に行われた行動が一覧表示されま
{% endif %}
{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-##### `team` カテゴリ
+#### `team` category actions
| アクション | 説明 |
| -------------------- | ---------------------------------------------------------------------------------------------------- |
@@ -385,60 +520,13 @@ Audit log には、過去 90 日間に行われた行動が一覧表示されま
| `remove_repository` | リポジトリが Team の管理下でなくなるときにトリガーされます。 |
{% endif %}
-##### `team_discussions` カテゴリ
+#### `team_discussions` category actions
| アクション | 説明 |
| --------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| `disable` | Organization のオーナーが Organization の Team ディスカッションを無効にするときにトリガーされます。 詳しい情報については [Organization の Team ディスカッションの無効化](/articles/disabling-team-discussions-for-your-organization)を参照してください。 |
| `enable` | Organization のオーナーが Organization の Team ディスカッションを有効にするときにトリガーされます。 |
-#### アクション時間に基づく検索
-
-`created` 修飾子を使用して、行われた日時に基づいて Audit log 内の行動をフィルタリングします。 {% data reusables.time_date.date_format %} {% data reusables.time_date.time_format %}
-
-{% data reusables.search.date_gt_lt %} 例:
-
- * `created:2014-07-08` は、2014 年 7 月 8 日に発生したイベントをすべて検索します。
- * `created:>=2014-07-08` は、2014 年 7 月 8 日かそれ以降に生じたすべてのイベントを検索します。
- * `created:<=2014-07-08`は、2014 年 7 月 8 日かそれ以前に生じたすべてのイベントを検索します。
- * `created:2014-07-01..2014-07-31`は、2014 年 7 月に起きたすべてのイベントを検索します。
-
-Audit log には過去 90 日間のデータが存在しますが、`created` 修飾子を使用すればそれより前のイベントを検索できます。
-
-#### 場所に基づく検索
-
-修飾子 `country` を使用すれば、発信元の国に基づいて Audit log 内の行動をフィルタリングできます。 国の 2 文字のショートコードまたはフル ネームを使用できます。 名前に空白がある国は引用符で囲む必要があることに注意してください。 例:
-
- * `country:de` は、ドイツで発生したイベントをすべて検索します。
- * `country:Mexico` はメキシコで発生したすべてのイベントを検索します。
- * `country:"United States"` はアメリカ合衆国で発生したすべてのイベントを検索します。
-
-{% if currentVersion == "free-pro-team@latest" %}
-### Audit log をエクスポートする
-
-{% data reusables.audit_log.export-log %}
-{% data reusables.audit_log.exported-log-keys-and-values %}
-{% endif %}
-
-### Audit log API を使用する
-
-{% note %}
-
-**メモ**: Audit log API は、{% data variables.product.prodname_enterprise %} を使用している Organization が利用できます。 {% data reusables.gated-features.more-info-org-products %}
-
-{% endnote %}
-
-IP の安全性を保ち、Organization のコンプライアンスを守るため、Audit log API を使って、Audit log データのコピーを保存し、モニタリングできます。
-* Organization またはリポジトリの設定へのアクセス
-* 権限の変更
-* Organization、リポジトリ、または Team におけるユーザの追加や削除
-* 管理者に昇格しているユーザ
-* GitHub App の権限の変更
-
-GraphQL のレスポンスには、90 日から 120 日までのデータを含めることができます。
-
-たとえば、GraphQL にリクエストして、Organization に新しく追加された Organization メンバー全員を表示できます。 詳細は「[GraphQL API Audit Log](/graphql/reference/interfaces#auditentry/)」を参照してください。
-
### 参考リンク
- "[Organization を安全に保つ](/articles/keeping-your-organization-secure)"
diff --git a/translations/ja-JP/content/github/setting-up-and-managing-your-enterprise/about-enterprise-accounts.md b/translations/ja-JP/content/github/setting-up-and-managing-your-enterprise/about-enterprise-accounts.md
index 22b31cae120e..51ecaa9ad0d4 100644
--- a/translations/ja-JP/content/github/setting-up-and-managing-your-enterprise/about-enterprise-accounts.md
+++ b/translations/ja-JP/content/github/setting-up-and-managing-your-enterprise/about-enterprise-accounts.md
@@ -20,7 +20,7 @@ Enterprise アカウントでは、複数の {% data variables.product.prodname_
- セキュリティ (シングルサインオン、2 要素認証)
- {% data variables.contact.enterprise_support %} とのリクエストおよび Support Bundle の共有
-{% data reusables.enterprise-accounts.enterprise-accounts-billing %}
+{% data reusables.enterprise-accounts.enterprise-accounts-billing %} For more information about managing your {% data variables.product.prodname_ghe_cloud %} subscription, see "[Viewing the subscription and usage for your enterprise account](/articles/viewing-the-subscription-and-usage-for-your-enterprise-account)." For more information about managing your {% data variables.product.prodname_ghe_server %} billing settings, see "[Managing billing for your enterprise](/admin/overview/managing-billing-for-your-enterprise)."
{% data variables.product.prodname_ghe_cloud %} と {% data variables.product.prodname_ghe_server %} の違いについては、「[{% data variables.product.prodname_dotcom %} の製品](/articles/githubs-products)」を参照してください。 {% data variables.product.prodname_enterprise %} にアップグレードする、または Enterprise アカウントを使い始める場合は、{% data variables.contact.contact_enterprise_sales %} にお問い合わせください。
diff --git a/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/about-your-profile.md b/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/about-your-profile.md
index 862054ed284e..71a93f02956a 100644
--- a/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/about-your-profile.md
+++ b/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/about-your-profile.md
@@ -13,7 +13,7 @@ versions:
以前の職場、コントリビュートしたプロジェクト、あなたが興味を持っていることなど、他者が知りたいあなたに関する個人情報を略歴に追加できます。 詳細は「[プロフィールに略歴を追加する](/articles/personalizing-your-profile/#adding-a-bio-to-your-profile)」を参照してください。
-{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
{% data reusables.profile.profile-readme %}
@@ -23,19 +23,17 @@ versions:
あなたのプロフィールにアクセスすると、あなたがオープンした Issue やプルリクエスト、行ったコミット、レビューしたプルリクエストなど、あなたのコントリビューションのアクティビティのタイムラインが表示されます。 パブリックコントリビューションだけを表示させることも、プライベートな匿名化されたコントリビューションも表示させることもできます。 詳細は「[プロフィールページ上にコントリビューションを表示する](/articles/viewing-contributions-on-your-profile-page)」または「[プライベートコントリビューションをプロフィールで公開または非公開にする](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)」を参照してください。
-以下の内容も表示されます:
+People who visit your profile can also see the following information.
-- あなたが所有している、もしくはコントリビューションしたリポジトリと Gist。 リポジトリおよび Gist をプロフィールにピン止めすることで、あなたの最も優れた作業を提示することができます。 詳細は「[プロフィールにアイテムをピン止めする](/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile)」を参照してください。
-- Star を付けたリポジトリ。 詳しい情報については、「[Star を付けてリポジトリを保存する](/articles/saving-repositories-with-stars/)」を参照してください。
-- あなたが最もアクティブな Organization、リポジトリ、Team でのあなたのアクティビティの概要。 For more information, see "[Showing an overview of your activity on your profile](/articles/showing-an-overview-of-your-activity-on-your-profile).{% if currentVersion == "free-pro-team@latest" %}
-- {% data variables.product.prodname_arctic_vault %}、{% data variables.product.prodname_sponsors %}、または {% data variables.product.company_short %} 開発者プログラムなどのプログラムに参加したことを示すバッジ。
-- {% data variables.product.prodname_pro %} を使っているか。 詳細は「[プロフィールをパーソナライズする](/articles/personalizing-your-profile)」を参照してください。{% endif %}
+- あなたが所有している、もしくはコントリビューションしたリポジトリと Gist。 {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}You can showcase your best work by pinning repositories and gists to your profile. 詳細は「[プロフィールにアイテムをピン止めする](/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile)」を参照してください。{% endif %}
+- Star を付けたリポジトリ。 For more information, see "[Saving repositories with stars](/articles/saving-repositories-with-stars/)."
+- あなたが最もアクティブな Organization、リポジトリ、Team でのあなたのアクティビティの概要。 For more information, see "[Showing an overview of your activity on your profile](/articles/showing-an-overview-of-your-activity-on-your-profile)."{% if currentVersion == "free-pro-team@latest" %}
+- Badges that show if you use {% data variables.product.prodname_pro %} or participate in programs like the {% data variables.product.prodname_arctic_vault %}, {% data variables.product.prodname_sponsors %}, or the {% data variables.product.company_short %} Developer Program. 詳細は「[プロフィールをパーソナライズする](/github/setting-up-and-managing-your-github-profile/personalizing-your-profile#displaying-badges-on-your-profile)」を参照してください。{% endif %}
You can also set a status on your profile to provide information about your availability. 詳細は「[ステータスを設定する](/articles/personalizing-your-profile/#setting-a-status)」を参照してください。
### 参考リンク
- [プロフィール画像のセットアップ方法](/articles/how-do-i-set-up-my-profile-picture)
-- [プロフィールへのリポジトリのピン止め](/articles/pinning-repositories-to-your-profile)
- [プロフィールでプライベートコントリビューションを公開または非表示にする](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)
- [プロフィール上でのコントリビューションの表示](/articles/viewing-contributions-on-your-profile)
diff --git a/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme.md b/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme.md
index c9cd864d1fe5..90f0e64bd799 100644
--- a/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme.md
+++ b/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme.md
@@ -4,7 +4,6 @@ intro: 'You can add a README to your {% data variables.product.prodname_dotcom %
versions:
free-pro-team: '*'
enterprise-server: '>=2.22'
- github-ae: '*'
---
### About your profile README
diff --git a/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/personalizing-your-profile.md b/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/personalizing-your-profile.md
index 08b5213c5474..f5aa5f5525a2 100644
--- a/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/personalizing-your-profile.md
+++ b/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/personalizing-your-profile.md
@@ -50,9 +50,9 @@ versions:
自分に関する情報を他の {% data variables.product.product_name %} ユーザーと共有するには、プロフィールに略歴を追加します。 [@メンション](/articles/basic-writing-and-formatting-syntax)と絵文字を使えば、あなたの現在あるいは過去の職場、職種、またどんな種類のコーヒーを飲んでいるかといった情報も含めることができます。
-{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
-自分に関するカスタマイズした情報を長いフォームで、もっと目立つように表示する場合は、プロフィール README を使用することもできます。 プロフィール README の詳細は、「[プロフィール README の管理](/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme)」を参照してください。
+自分に関するカスタマイズした情報を長いフォームで、もっと目立つように表示する場合は、プロフィール README を使用することもできます。 For more information, see "[Managing your profile README](/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme)."
{% endif %}
diff --git a/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile.md b/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile.md
index 1326e0743718..5fdf48300275 100644
--- a/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile.md
+++ b/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile.md
@@ -7,7 +7,6 @@ redirect_from:
versions:
free-pro-team: '*'
enterprise-server: '*'
- github-ae: '*'
---
あなたが所有するか、コントリビュートしたパブリックリポジトリをピン止めできます。 フォークへのコミットはコントリビューションとして扱われないので、所有していないフォークをピン止めすることはできません。 詳細は「[プロフィール上でコントリビューションが表示されない理由](/articles/why-are-my-contributions-not-showing-up-on-my-profile)」を参照してください。
diff --git a/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/publicizing-or-hiding-your-private-contributions-on-your-profile.md b/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/publicizing-or-hiding-your-private-contributions-on-your-profile.md
index 1f990abd3b60..eabd9dc7d6f4 100644
--- a/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/publicizing-or-hiding-your-private-contributions-on-your-profile.md
+++ b/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/publicizing-or-hiding-your-private-contributions-on-your-profile.md
@@ -1,6 +1,6 @@
---
title: プライベートコントリビューションをプロフィールで公開または非公開にする
-intro: '{% data variables.product.product_name %} プロフィールには、過去 1 年間のリポジトリコントリビューションのグラフが表示されます。 パブリックリポジトリのアクティビティを表示するほか、プライベートリポジトリからのアクティビティも匿名化して表示することができます。'
+intro: '{% data variables.product.product_name %} プロフィールには、過去 1 年間のリポジトリコントリビューションのグラフが表示されます。 You can choose to show anonymized activity from {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" %}private and internal{% else %}private{% endif %} repositories{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %} in addition to the activity from public repositories{% endif %}.'
redirect_from:
- /articles/publicizing-or-hiding-your-private-contributions-on-your-profile
versions:
diff --git a/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/viewing-contributions-on-your-profile.md b/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/viewing-contributions-on-your-profile.md
index c393f42a3fe4..66d16e269582 100644
--- a/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/viewing-contributions-on-your-profile.md
+++ b/translations/ja-JP/content/github/setting-up-and-managing-your-github-profile/viewing-contributions-on-your-profile.md
@@ -1,6 +1,6 @@
---
title: プロフィールでコントリビューションを表示する
-intro: '{% data variables.product.product_name %} プロフィールは、固定されたリポジトリと過去 1 年間のリポジトリコントリビューション貢献のグラフを表示します。'
+intro: 'Your {% data variables.product.product_name %} profile shows off {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}your pinned repositories as well as{% endif %} a graph of your repository contributions over the past year.'
redirect_from:
- /articles/viewing-contributions/
- /articles/viewing-contributions-on-your-profile-page/
@@ -11,7 +11,7 @@ versions:
github-ae: '*'
---
-コントリビューショングラフは、パブリックリポジトリからのアクティビティを表示します。 パブリックリポジトリとプライベートリポジトリの両方からアクティビティを表示するように選択できます。プライベートリポジトリでは、アクティビティの詳細を表示できます。 詳細は「[プライベートコントリビューションをプロフィールで公開または非公開にする](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)」を参照してください。
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}Your contribution graph shows activity from public repositories. {% endif %}You can choose to show activity from {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}both public and{% endif %}private repositories, with specific details of your activity in private repositories anonymized. 詳細は「[プライベートコントリビューションをプロフィールで公開または非公開にする](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)」を参照してください。
{% note %}
@@ -33,16 +33,20 @@ versions:
### 人気のあるリポジトリ
-このセクションには、ウォッチャーが最も多いリポジトリが表示されます。 [自分のプロフィールにリポジトリをピン止めする](/articles/pinning-repositories-to-your-profile)と、このセクションは [Pinned repositories] に変わります。
+このセクションには、ウォッチャーが最も多いリポジトリが表示されます。 {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}Once you [pin repositories to your profile](/articles/pinning-repositories-to-your-profile), this section will change to "Pinned repositories."{% endif %}

+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
### Pinned repositories
このセクションには最大 6 つのパブリックリポジトリが表示されます。このリポジトリには、自分のリポジトリだけでなく、自分がコントリビュートしたリポジトリも含めることができます。 選択したリポジトリに関する重要な詳細を簡単に見るために、このセクションの各リポジトリには、行われている作業の要約、そのリポジトリに付いた [Star](/articles/saving-repositories-with-stars/) の数、およびそのリポジトリで使用されている主なプログラミング言語が含まれます。 詳細は「[プロフィールにリポジトリをピン止めする](/articles/pinning-repositories-to-your-profile)」を参照してください。

+{% endif %}
+
### コントリビューションカレンダー
コントリビューションカレンダーは、コントリビューションアクティビティを表示します。
diff --git a/translations/ja-JP/content/github/setting-up-and-managing-your-github-user-account/about-your-personal-dashboard.md b/translations/ja-JP/content/github/setting-up-and-managing-your-github-user-account/about-your-personal-dashboard.md
index 3f43666a0914..cb615c8d6cbf 100644
--- a/translations/ja-JP/content/github/setting-up-and-managing-your-github-user-account/about-your-personal-dashboard.md
+++ b/translations/ja-JP/content/github/setting-up-and-managing-your-github-user-account/about-your-personal-dashboard.md
@@ -15,7 +15,7 @@ versions:
パーソナルダッシュボードは、{% data variables.product.product_name %}にサインインしたときに最初に表示されるページです。
-サインインした後にパーソナルダッシュボードにアクセスするには、{% data variables.product.product_url %} の任意のページの左上の隅にある {% octicon "mark-github" aria-label="The github octocat logo" %} をクリックします。
+サインインした後にパーソナルダッシュボードにアクセスするには、{% data variables.product.product_name %} の任意のページの左上の隅にある {% octicon "mark-github" aria-label="The github octocat logo" %} をクリックします。
### 最近のアクティビティを見つける
@@ -39,11 +39,11 @@ The list of top repositories is automatically generated, and can include any rep
ニュースフィードでは、あなたがフォローしているユーザが以下のことをした場合に更新情報が示されます:
- リポジトリに Star を付ける。
-- 他のユーザをフォローする。
-- パブリックリポジトリを作成
+- Follows another user.{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- Creates a public repository.{% endif %}
- あなたが Watch しているリポジトリ上で "help wanted" あるいは "good first issue" のラベルを付けた Issue あるいはプルリクエストをオープンする。
-- あなたが Watch しているリポジトリにコミットをプッシュする。
-- パブリックリポジトリをフォークする。
+- Pushes commits to a repository you watch.{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- Forks a public repository.{% endif %}
リポジトリへの Star 付けや人のフォローに関する詳細は「[Star を付けてリポジトリを保存する](/articles/saving-repositories-with-stars/)」および「[人をフォローする](/articles/following-people)」を参照してください。
diff --git a/translations/ja-JP/content/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository.md b/translations/ja-JP/content/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository.md
index b78dc072407a..0bdabd611ab2 100644
--- a/translations/ja-JP/content/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository.md
+++ b/translations/ja-JP/content/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository.md
@@ -50,7 +50,7 @@ Organization が所有するリポジトリは、細やかなアクセスを許
### 参考リンク
-- [ユーザ アカウントのリポジトリ権限レベル](/articles/permission-levels-for-a-user-account-repository/#collaborator-access-on-a-repository-owned-by-a-user-account)
+- "[ユーザ アカウントリポジトリの権限レベル](/articles/permission-levels-for-a-user-account-repository/#collaborator-access-for-a-repository-owned-by-a-user-account)"
- [個人リポジトリからコラボレーターを削除する](/articles/removing-a-collaborator-from-a-personal-repository)
- [コラボレーターのリポジトリから自分を削除する](/articles/removing-yourself-from-a-collaborator-s-repository)
- [メンバーを Team に編成する](/articles/organizing-members-into-teams)
diff --git a/translations/ja-JP/content/github/setting-up-and-managing-your-github-user-account/permission-levels-for-a-user-account-repository.md b/translations/ja-JP/content/github/setting-up-and-managing-your-github-user-account/permission-levels-for-a-user-account-repository.md
index bd7d09f9239b..e05588ea6773 100644
--- a/translations/ja-JP/content/github/setting-up-and-managing-your-github-user-account/permission-levels-for-a-user-account-repository.md
+++ b/translations/ja-JP/content/github/setting-up-and-managing-your-github-user-account/permission-levels-for-a-user-account-repository.md
@@ -1,6 +1,6 @@
---
title: ユーザーアカウントのリポジトリ権限レベル
-intro: 'ユーザーアカウントが所有するリポジトリは、*リポジトリオーナー*と*コラボレーター*という 2 つの権限レベルを持ちます。'
+intro: 'A repository owned by a user account has two permission levels: the repository owner and collaborators.'
redirect_from:
- /articles/permission-levels-for-a-user-account-repository
versions:
@@ -9,38 +9,48 @@ versions:
github-ae: '*'
---
+### About permissions levels for a user account repository
+
+Repositories owned by user accounts have one owner. Ownership permissions can't be shared with another user account.
+
+You can also {% if currentVersion == "free-pro-team@latest" %}invite{% else %}add{% endif %} users on {% data variables.product.product_name %} to your repository as collaborators. For more information, see "[Inviting collaborators to a personal repository](/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository)."
+
{% tip %}
-**ヒント:** ユーザーアカウントが所有しているリポジトリに対して、より精細な読み取り/書き込みアクセス権が必要な場合には、リポジトリを Organization に移譲することを検討してください。 詳細は「[リポジトリを移譲する](/articles/transferring-a-repository)」を参照してください。
+**Tip:** If you require more granular access to a repository owned by your user account, consider transferring the repository to an organization. 詳細は「[リポジトリを移譲する](/github/administering-a-repository/transferring-a-repository#transferring-a-repository-owned-by-your-user-account)」を参照してください。
{% endtip %}
-#### ユーザーアカウントが所有しているリポジトリに対するオーナーアクセス権
-
-リポジトリオーナーは、リポジトリを完全に制御することができます。 リポジトリコラボレータによって許可されるすべての権限に加えて、リポジトリオーナーは次の操作が可能です:
-
-- {% if currentVersion == "free-pro-team@latest" %}[Invite collaborators](/articles/inviting-collaborators-to-a-personal-repository){% else %}[Add collaborators](/articles/inviting-collaborators-to-a-personal-repository){% endif %}
-- Change the visibility of the repository (from [public to private](/articles/making-a-public-repository-private), or from [private to public](/articles/making-a-private-repository-public)){% if currentVersion == "free-pro-team@latest" %}
-- [リポジトリでのインタラクションを制限する](/articles/limiting-interactions-with-your-repository){% endif %}
-- 保護されたブランチで、レビューの承認がなくてもプルリクエストをマージする
-- [リポジトリを削除する](/articles/deleting-a-repository)
-- [Manage a repository's topics](/articles/classifying-your-repository-with-topics){% if currentVersion == "free-pro-team@latest" %}
-- Manage security and analysis settings. For more information, see "[Managing security and analysis settings for your user account](/github/setting-up-and-managing-your-github-user-account/managing-security-and-analysis-settings-for-your-user-account)."{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-- [Enable the dependency graph](/github/visualizing-repository-data-with-graphs/exploring-the-dependencies-and-dependents-of-a-repository) for a private repository{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-- パッケージを削除する。 詳細は「[>パッケージを削除する](/github/managing-packages-with-github-packages/deleting-a-package)」を参照してください。{% endif %}
-- リポジトリソーシャルカードを作成および編集する。 詳細は「[リポジトリのソーシャルメディア向けプレビューをカスタマイズする](/articles/customizing-your-repositorys-social-media-preview)」を参照してください。
-- リポジトリをテンプレートにする。 For more information, see "[Creating a template repository](/articles/creating-a-template-repository)."{% if currentVersion != "github-ae@latest" %}
-- Receive [{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot_alerts %}{% else %}security alerts{% endif %} for vulnerable dependencies](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies) in a repository.{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-- リポジトリで {% data variables.product.prodname_dependabot_alerts %} を閉じます。 詳細については、「[リポジトリ内の脆弱な依存関係を表示・更新する](/github/managing-security-vulnerabilities/viewing-and-updating-vulnerable-dependencies-in-your-repository)」を参照してください。
-- [プライベートリポジトリのデータ使用を管理する](/github/understanding-how-github-uses-and-protects-your-data/managing-data-use-settings-for-your-private-repository){% endif %}
-- [リポジトリのコードオーナーを定義する](/articles/about-code-owners)
-- [Archive repositories](/articles/about-archiving-repositories){% if currentVersion == "free-pro-team@latest" %}
-- セキュリティアドバイザリを作成する。 詳しい情報については「[{% data variables.product.prodname_security_advisories %}について](/github/managing-security-vulnerabilities/about-github-security-advisories)」を参照してください。
-- スポンサーボタンを表示する。 詳細は「[リポジトリにスポンサーボタンを表示する](/articles/displaying-a-sponsor-button-in-your-repository)」を参照してください。{% endif %}
-
-ユーザアカウントが所有するリポジトリの**オーナーは 1 人**だけです。この権限を他のユーザアカウントと共有することはできません。 リポジトリの所有権を他のユーザに委譲するには、「[リポジトリを委譲する方法](/articles/how-to-transfer-a-repository)」を参照してください。
-
-#### ユーザーアカウントが所有しているリポジトリに対するコラボレーターアクセス権
+### Owner access for a repository owned by a user account
+
+リポジトリオーナーは、リポジトリを完全に制御することができます。 In addition to the actions that any collaborator can perform, the repository owner can perform the following actions.
+
+| アクション | 詳細情報 |
+|:---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |:----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| {% if currentVersion == "free-pro-team@latest" %}Invite collaborators{% else %}Add collaborators{% endif %} | |
+| [個人リポジトリへのコラボレータの招待](/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository) | |
+| Change the visibility of the repository | "[Setting repository visibility](/github/administering-a-repository/setting-repository-visibility)" |{% if currentVersion == "free-pro-team@latest" %}
+| Limit interactions with the repository | "[Limiting interactions in your repository](/github/building-a-strong-community/limiting-interactions-in-your-repository)" |{% endif %}
+| 保護されたブランチで、レビューの承認がなくてもプルリクエストをマージする | [保護されたブランチについて](/github/administering-a-repository/about-protected-branches) |
+| リポジトリを削除する | 「[リポジトリを削除する](/github/administering-a-repository/deleting-a-repository)」 |
+| Manage the repository's topics | "[Classifying your repository with topics](/github/administering-a-repository/classifying-your-repository-with-topics)" |{% if currentVersion == "free-pro-team@latest" %}
+| Manage security and analysis settings for the repository | "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository)" |{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| Enable the dependency graph for a private repository | "[Exploring the dependencies of a repository](/github/visualizing-repository-data-with-graphs/exploring-the-dependencies-of-a-repository#enabling-and-disabling-the-dependency-graph-for-a-private-repository)" |{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| パッケージの削除 | "[Deleting a package](/github/managing-packages-with-github-packages/deleting-a-package)" |{% endif %}
+| Customize the repository's social media preview | "[Customizing your repository's social media preview](/github/administering-a-repository/customizing-your-repositorys-social-media-preview)" |
+| Create a template from the repository | "[Creating a template repository](/github/creating-cloning-and-archiving-repositories/creating-a-template-repository)" |{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+| Receive | |
+| {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot_alerts %}{% else %}security alerts{% endif %} for vulnerable dependencies | "[About alerts for vulnerable dependencies](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies)" |{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| Dismiss {% data variables.product.prodname_dependabot_alerts %} in the repository | [リポジトリ内の脆弱な依存関係を表示・更新する](/github/managing-security-vulnerabilities/viewing-and-updating-vulnerable-dependencies-in-your-repository) |
+| Manage data use for a private repository | "[Managing data use settings for your private repository](/github/understanding-how-github-uses-and-protects-your-data/managing-data-use-settings-for-your-private-repository)"|{% endif %}
+| リポジトリのコードオーナーを定義する | 「[コードオーナー'について](/github/creating-cloning-and-archiving-repositories/about-code-owners)」 |
+| Archive the repository | "[About archiving repositories](/github/creating-cloning-and-archiving-repositories/about-archiving-repositories)" |{% if currentVersion == "free-pro-team@latest" %}
+| Create security advisories | "[About {% data variables.product.prodname_security_advisories %}](/github/managing-security-vulnerabilities/about-github-security-advisories)" |
+| Display a sponsor button | "[Displaying a sponsor button in your repository](/github/administering-a-repository/displaying-a-sponsor-button-in-your-repository)" |{% endif %}
+
+### Collaborator access for a repository owned by a user account
+
+Collaborators on a personal repository can pull (read) the contents of the repository and push (write) changes to the repository.
{% note %}
@@ -48,27 +58,26 @@ versions:
{% endnote %}
-個人リポジトリでのコラボレーターは、次の操作が可能です:
-
-- リポジトリに対してプッシュする (書き込む)、プル (読み取る)、フォーク (コピーする)
-- ラベルとマイルストーンを作成、適用、削除する
-- Issue をオープン、再オープン、割り当てする
-- コミット、プルリクエスト、Issue に対するコメントを編集および削除する
-- Issue またはプルリクエストを重複としてマークする。 詳細は「[重複した Issue やプルリクエストについて](/articles/about-duplicate-issues-and-pull-requests)」を参照してください。
-- Open, merge and close pull requests
-- 提案された変更をプルリクエストに適用する。 詳細は「[プルリクエストでのフィードバックを取り込む](/articles/incorporating-feedback-in-your-pull-request)」を参照してください。
-- Send pull requests from forks of the repository{% if currentVersion == "free-pro-team@latest" %}
-- パッケージを公開、表示、インストールする。 詳細は、「[パッケージの公開と管理](/github/managing-packages-with-github-packages/publishing-and-managing-packages)」を参照してください。{% endif %}
-- ウィキを作成および編集する
-- リリースの作成と編集。 詳細は「[リポジトリのリリースを管理する](/github/administering-a-repository/managing-releases-in-a-repository)」を参照してください。
-- リポジトリでコラボレーターである自身を削除する
-- マージ可能性に影響するプルリクエストレビューをサブミットする
-- リポジトリに指定されたコードオーナーとして行動する。 詳細は「[コードオーナーについて](/articles/about-code-owners)」を参照してください。
-- 会話をロックする。 For more information, see "[Locking conversations](/articles/locking-conversations)."{% if currentVersion == "free-pro-team@latest" %}
-- 乱用コンテンツを {% data variables.contact.contact_support %} にレポートする 詳細は「[乱用やスパムをレポートする](/articles/reporting-abuse-or-spam)」を参照してください。{% endif %}
-- 他のリポジトリへ Issue を移譲する。 詳細は「[他のリポジトリへ Issue を移譲する](/articles/transferring-an-issue-to-another-repository)」を参照してください。
+Collaborators can also perform the following actions.
+
+| アクション | 詳細情報 |
+|:----------------------------------------------------------------------------------------- |:------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| Fork the repository | 「[フォークについて](/github/collaborating-with-issues-and-pull-requests/about-forks)」 |
+| Create, edit, and delete comments on commits, pull requests, and issues in the repository | - "[About issues](/github/managing-your-work-on-github/about-issues)"
- "[Commenting on a pull request](/github/collaborating-with-issues-and-pull-requests/commenting-on-a-pull-request)"
- "[Managing disruptive comments](/github/building-a-strong-community/managing-disruptive-comments)"
|
+| Create, assign, close, and re-open issues in the repository | "[Managing your work with issues](/github/managing-your-work-on-github/managing-your-work-with-issues)" |
+| Manage labels for issues and pull requests in the repository | "[Labeling issues and pull requests](/github/managing-your-work-on-github/labeling-issues-and-pull-requests)" |
+| Manage milestones for issues and pull requests in the repository | [Issueやプルリクエストのためのマイルストーンの作成と編集](/github/managing-your-work-on-github/creating-and-editing-milestones-for-issues-and-pull-requests) |
+| Mark an issue or pull request in the repository as a duplicate | "[About duplicate issues and pull requests](/github/managing-your-work-on-github/about-duplicate-issues-and-pull-requests)" |
+| Create, merge, and close pull requests in the repository | "[Proposing changes to your work with pull requests](/github/collaborating-with-issues-and-pull-requests/proposing-changes-to-your-work-with-pull-requests)" |
+| Apply suggested changes to pull requests in the repository | "[Incorporating feedback in your pull request](/github/collaborating-with-issues-and-pull-requests/incorporating-feedback-in-your-pull-request)" |
+| Create a pull request from a fork of the repository | [フォークからプルリクエストを作成する](/github/collaborating-with-issues-and-pull-requests/creating-a-pull-request-from-a-fork) |
+| Submit a review on a pull request that affects the mergeability of the pull request | 「[プルリクエストで提案された変更をレビューする](/github/collaborating-with-issues-and-pull-requests/reviewing-proposed-changes-in-a-pull-request)」 |
+| Create and edit a wiki for the repository | 「[ウィキについて](/github/building-a-strong-community/about-wikis)」 |
+| Create and edit releases for the repository | "[Managing releases in a repository](/github/administering-a-repository/managing-releases-in-a-repository)" |
+| Act as a code owner for the repository | "[About code owners](/articles/about-code-owners)" |{% if currentVersion == "free-pro-team@latest" %}
+| Publish, view, or install packages | "[Publishing and managing packages](/github/managing-packages-with-github-packages/publishing-and-managing-packages)" |{% endif %}
+| リポジトリでコラボレーターである自身を削除する | [コラボレーターのリポジトリから自分を削除する](/github/setting-up-and-managing-your-github-user-account/removing-yourself-from-a-collaborators-repository) |
### 参考リンク
-- [個人リポジトリへのコラボレータの招待](/articles/inviting-collaborators-to-a-personal-repository)
- [Organization のリポジトリ権限レベル](/articles/repository-permission-levels-for-an-organization)
diff --git a/translations/ja-JP/content/github/site-policy/github-marketplace-terms-of-service.md b/translations/ja-JP/content/github/site-policy/github-marketplace-terms-of-service.md
index ce8b82561e6b..bc0b7126cd13 100644
--- a/translations/ja-JP/content/github/site-policy/github-marketplace-terms-of-service.md
+++ b/translations/ja-JP/content/github/site-policy/github-marketplace-terms-of-service.md
@@ -6,11 +6,11 @@ versions:
free-pro-team: '*'
---
-GitHub Marketplace (「Marketplace」) へようこそ! また、こちらのページをご覧いただきありがとうございます。 GitHub Marketplaceにアクセスしたり、利用したりする前に、この利用規約 (「Marketplace利用規約」) をよくお読みください。 GitHub Marketplaceは、GitHub.comアカウントで利用できる「開発者製品」を無料で入手または有料で購入できるプラットフォームです。 Although sold by GitHub, Inc. ("GitHub", "we", "us"), Developer Products may be developed and maintained by either GitHub or by third-party software providers, and may require you to agree to a separate terms of service. 「開発者製品」の購入や利用には、本「Marketplace利用規約」が適用され、該当する料金が課されます。また、「開発者製品」のサードパーティーライセンサー(「製品提供者」)による利用規約が追加で適用される場合もあります。
+GitHub Marketplace (「Marketplace」) へようこそ! また、こちらのページをご覧いただきありがとうございます。 GitHub Marketplaceにアクセスしたり、利用したりする前に、この利用規約 (「Marketplace利用規約」) をよくお読みください。 GitHub Marketplace is a platform that allows you to select developer apps or actions (for free or for a charge) that can be used with your GitHub.com account ("Developer Products"). Although offered by GitHub, Inc. ("GitHub", "we", "us"), Developer Products may be developed and maintained by either GitHub or by third-party software providers. Your selection or use of Developer Products is subject to these Marketplace Terms and any applicable fees, and may require you to agree to additional terms as provided by the third party licensor of that Developer Product (the "Product Provider").
「Marketplace」を利用することにより、お客様は本「Marketplace利用規約」に従うことに同意するものとします。
-発効日: 2017年10月11日
+Effective Date: November 20, 2020
### A. GitHub.com利用規約
@@ -40,11 +40,11 @@ GitHub Marketplace (「Marketplace」) へようこそ! また、こちらの
### E. お客様のデータとGitHubのプライバシーポリシー
-**プライバシー。**「開発者製品」を購入またはサブスクライブする際、「開発者製品」をお客様に提供するため、お客様のプライバシー設定に関わらず、GitHubは(「[GitHub のプライバシーについての声明](/articles/github-privacy-statement/)」の定義による) お客様の特定の「個人情報」を「製品提供者」に対して共有する必要があります。 GitHub は、お客様が選択した「開発者製品」の要件に応じて、ユーザアカウント名、ID、プライマリメールアドレスといった最低限の情報から、プライベートデータの読み取りや変更を含む、リポジトリ内のコンテンツに対するアクセスなどに至るまで共有することができます。 お客様は OAuth を通じて権限を付与する際に、「開発者製品」が要求する権限のスコープを確認し、承認または拒否できます。 [GitHub のプライバシーについての声明](/articles/github-privacy-statement/)に従い、「製品提供者」には取引のために必要な最小限の情報のみを提供します。
+**Privacy.** When you select or use a Developer Product, GitHub may share certain Personal Information (as defined in the [GitHub Privacy Statement](/articles/github-privacy-statement/)) with the Product Provider (if any such Personal Information is received from you) in order to provide you with the Developer Product, regardless of your privacy settings. GitHub は、お客様が選択した「開発者製品」の要件に応じて、ユーザアカウント名、ID、プライマリメールアドレスといった最低限の情報から、プライベートデータの読み取りや変更を含む、リポジトリ内のコンテンツに対するアクセスなどに至るまで共有することができます。 お客様は OAuth を通じて権限を付与する際に、「開発者製品」が要求する権限のスコープを確認し、承認または拒否できます。
-「開発者製品」のサービスをキャンセルし、アカウント設定を通じてアクセスを取り消すと、「製品提供者」はお客様のアカウントにアクセスできなくなります。 「製品提供者」は、定められた期間内にシステムからお客様の「個人情報」を削除する責任を負います。 お客様のアカウントが正しく解約されたことを確認するため、「製品提供者」にご連絡ください。
+If you cease using the Developer Product and revoke access through your account settings, the Product Provider will no longer be able to access your account. 「製品提供者」は、定められた期間内にシステムからお客様の「個人情報」を削除する責任を負います。 お客様のアカウントが正しく解約されたことを確認するため、「製品提供者」にご連絡ください。
-**データ セキュリティの免責事項。**「開発者製品」を購入またはサブスクライブする際、該当「開発者製品」のセキュリティおよびお客様のデータに関する管理については、その責任を「製品提供者」が負います。 お客様のセキュリティ、リスク、および法令遵守のため、「開発者製品」の購入および利用に際して、お客様はセキュリティの留意事項を理解する責任を負います。
+**Data Security and Privacy Disclaimer.** When you select or use a Developer Product, the security of the Developer Product and the custodianship of your data, including your Personal Information (if any), is the responsibility of the Product Provider. You are responsible for understanding the security and privacy considerations of the selection or use of the Developer Product for your own security and privacy risk and compliance considerations.
### F. 開発者製品に対する権利
diff --git a/translations/ja-JP/content/github/using-git/setting-your-username-in-git.md b/translations/ja-JP/content/github/using-git/setting-your-username-in-git.md
index 650cad75b4c9..53bfc598b9b1 100644
--- a/translations/ja-JP/content/github/using-git/setting-your-username-in-git.md
+++ b/translations/ja-JP/content/github/using-git/setting-your-username-in-git.md
@@ -20,13 +20,13 @@ versions:
2. {% data reusables.user_settings.set_your_git_username %}
```shell
$ git config --global user.name "Mona Lisa"
- ```
+ ```
3. {% data reusables.user_settings.confirm_git_username_correct %}
```shell
$ git config --global user.name
> Mona Lisa
- ```
+ ```
### 単一のリポジトリ用に Git ユーザ名を設定する
@@ -37,13 +37,13 @@ versions:
3. {% data reusables.user_settings.set_your_git_username %}
```shell
$ git config user.name "Mona Lisa"
- ```
+ ```
3. {% data reusables.user_settings.confirm_git_username_correct %}
```shell
$ git config user.name
> Mona Lisa
- ```
+ ```
### 参考リンク
diff --git a/translations/ja-JP/content/github/using-git/which-remote-url-should-i-use.md b/translations/ja-JP/content/github/using-git/which-remote-url-should-i-use.md
index 47015e8437da..9bbc4f1619fb 100644
--- a/translations/ja-JP/content/github/using-git/which-remote-url-should-i-use.md
+++ b/translations/ja-JP/content/github/using-git/which-remote-url-should-i-use.md
@@ -3,7 +3,7 @@ title: どのリモート URL を使うべきですか?
redirect_from:
- /articles/which-url-should-i-use/
- /articles/which-remote-url-should-i-use
-intro: '{% data variables.product.prodname_dotcom %} で使用できるリポジトリを複製する方法は複数あります。'
+intro: '{% data variables.product.product_location %} で使用できるリポジトリを複製する方法は複数あります。'
versions:
free-pro-team: '*'
enterprise-server: '*'
@@ -16,7 +16,7 @@ versions:
### Cloning with HTTPS URLs
-`https://` クローン URL は、パブリックおよびプライベートのすべてのリポジトリで利用できます。 これらの URL は、ファイアウォールまたはプロキシの内側にいる場合でも機能します。
+The `https://` clone URLs are available on all repositories, regardless of visibility. `https://` clone URLs work even if you are behind a firewall or proxy.
コマンドラインで、HTTPS URL を使用してリモートリポジトリに `git clone`、`git fetch`、`git pull` または `git push` を行った場合、{% data variables.product.product_name %} のユーザ名とパスワードの入力を求められます。 {% data reusables.user_settings.password-authentication-deprecation %}
diff --git a/translations/ja-JP/content/github/writing-on-github/creating-gists.md b/translations/ja-JP/content/github/writing-on-github/creating-gists.md
index 9e33f76c4344..a9e881d292ce 100644
--- a/translations/ja-JP/content/github/writing-on-github/creating-gists.md
+++ b/translations/ja-JP/content/github/writing-on-github/creating-gists.md
@@ -33,8 +33,12 @@ Secret gists don't show up in {% data variables.gists.discover_url %} and are no
- 誰かがあなたを Gist 中でメンションした場合。
- いずれかの Gist の上部で [** Subscribe**] をクリックして、Gist をサブスクライブした場合。
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
You can pin gists to your profile so other people can see them easily. 詳しい情報については、「[プロフィールにアイテムをピン止めする](/articles/pinning-items-to-your-profile)」を参照してください。
+{% endif %}
+
他の人が作成した Gist は、{% data variables.gists.gist_homepage %} にアクセスして [**All Gists**] をクリックすることで見つけることができます。 こうすると、すべての Gist が作成時刻または更新時刻でソートされて表示されるページに行きます。 また、Gist は {% data variables.gists.gist_search_url %} で言語ごとに検索できます。 Gist 検索は[コード検索](/articles/searching-code)と同じ検索構文を使います。
Gist は Git リポジトリであるため、完全なコミット履歴を diff とともに表示させることができます。 Gist はフォークしたりクローンしたりすることもできます。 詳細は「[Gist のフォークおよびクローン](/articles/forking-and-cloning-gists)」を参照してください。
diff --git a/translations/ja-JP/content/packages/managing-container-images-with-github-container-registry/deleting-a-container-image.md b/translations/ja-JP/content/packages/managing-container-images-with-github-container-registry/deleting-a-container-image.md
index e795078ea3d0..ff244bf0209f 100644
--- a/translations/ja-JP/content/packages/managing-container-images-with-github-container-registry/deleting-a-container-image.md
+++ b/translations/ja-JP/content/packages/managing-container-images-with-github-container-registry/deleting-a-container-image.md
@@ -16,10 +16,6 @@ versions:
パブリックパッケージを削除する場合、そのパッケージに依存するプロジェクトを破壊する可能性があることに注意してください。
-### 予約されているパッケージのバージョンと名前
-
-{% data reusables.package_registry.package-immutability %}
-
### {% data variables.product.prodname_dotcom %} 上でユーザが所持するコンテナイメージのバージョンを削除する
{% data reusables.package_registry.package-settings-from-user-level %}
diff --git a/translations/ja-JP/content/packages/publishing-and-managing-packages/deleting-a-package.md b/translations/ja-JP/content/packages/publishing-and-managing-packages/deleting-a-package.md
index a7187a2ec16a..2e680e34200c 100644
--- a/translations/ja-JP/content/packages/publishing-and-managing-packages/deleting-a-package.md
+++ b/translations/ja-JP/content/packages/publishing-and-managing-packages/deleting-a-package.md
@@ -35,10 +35,6 @@ At this time, {% data variables.product.prodname_registry %} on {% data variable
{% endif %}
-### 予約されているパッケージのバージョンと名前
-
-{% data reusables.package_registry.package-immutability %}
-
### {% data variables.product.product_name %}上でのプライベートパッケージのバージョンの削除
プライベートパッケージのバージョンを削除するには、そのリポジトリの管理権限が必要です。
diff --git a/translations/ja-JP/content/packages/publishing-and-managing-packages/publishing-a-package.md b/translations/ja-JP/content/packages/publishing-and-managing-packages/publishing-a-package.md
index 31c6a58a9560..b0f8b2cb2936 100644
--- a/translations/ja-JP/content/packages/publishing-and-managing-packages/publishing-a-package.md
+++ b/translations/ja-JP/content/packages/publishing-and-managing-packages/publishing-a-package.md
@@ -18,8 +18,6 @@ versions:
{% data reusables.package_registry.public-or-private-packages %} リポジトリには複数のパッケージを含めることができます。 混乱を避けるため、READMEと説明で各パッケージに関する情報を明確に提供してください。
-{% data reusables.package_registry.package-immutability %}
-
{% if currentVersion == "free-pro-team@latest" %}
新しいバージョンのパッケージでセキュリティの脆弱性が解決される場合は、リポジトリでセキュリティアドバイザリを公開する必要があります。
{% data variables.product.prodname_dotcom %} reviews each published security advisory and may use it to send {% data variables.product.prodname_dependabot_alerts %} to affected repositories. 詳しい情報については、「[GitHub セキュリティアドバイザリについて](/github/managing-security-vulnerabilities/about-github-security-advisories)」 を参照してください。
diff --git a/translations/ja-JP/content/packages/using-github-packages-with-your-projects-ecosystem/configuring-npm-for-use-with-github-packages.md b/translations/ja-JP/content/packages/using-github-packages-with-your-projects-ecosystem/configuring-npm-for-use-with-github-packages.md
index 7503988f82cb..3c1b67f34287 100644
--- a/translations/ja-JP/content/packages/using-github-packages-with-your-projects-ecosystem/configuring-npm-for-use-with-github-packages.md
+++ b/translations/ja-JP/content/packages/using-github-packages-with-your-projects-ecosystem/configuring-npm-for-use-with-github-packages.md
@@ -48,12 +48,15 @@ $ npm login --registry=https://npm.pkg.github.com
npmにログインすることで認証を受けるには、`npm login`コマンドを使ってください。*USERNAME*は{% data variables.product.prodname_dotcom %}のユーザ名で、*TOKEN*は個人アクセストークンで、*PUBLIC-EMAIL-ADDRESS*はメールアドレスで置き換えてください。
+If {% data variables.product.prodname_registry %} is not your default package registry for using npm and you want to use the `npm audit` command, we recommend you use the `--scope` flag with the owner of the package when you authenticate to {% data variables.product.prodname_registry %}.
+
{% if enterpriseServerVersions contains currentVersion %}
パッケージの作成に関する詳しい情報については[maven.apache.orgのドキュメンテーション](https://maven.apache.org/guides/getting-started/maven-in-five-minutes.html)を参照してください。
{% endif %}
```shell
-$ npm login --registry=https://{% if currentVersion == "free-pro-team@latest" %}npm.pkg.github.com{% else %}npm.HOSTNAME/{% endif %}
+$ npm login --scope=@OWNER --registry=https://{% if currentVersion == "free-pro-team@latest" %}npm.pkg.github.com{% else %}npm.HOSTNAME/{% endif %}
+
> Username: USERNAME
> Password: TOKEN
> Email: PUBLIC-EMAIL-ADDRESS
@@ -63,9 +66,10 @@ $ npm login --registry=https://{% if currentVersion == "free-pro-team@latest" %}
たとえば、以下の*OctodogApp*と*OctocatApp*は同じリポジトリに公開されます。
```shell
-registry=https://npm.pkg.github.com/OWNER
-@OWNER:registry=https://npm.pkg.github.com
-@OWNER:registry=https://npm.pkg.github.com
+$ npm login --scope=@OWNER --registry=https://HOSTNAME/_registry/npm/
+> Username: USERNAME
+> Password: TOKEN
+> Email: PUBLIC-EMAIL-ADDRESS
```
{% endif %}
diff --git a/translations/ja-JP/data/reusables/enterprise-accounts/enterprise-accounts-billing.md b/translations/ja-JP/data/reusables/enterprise-accounts/enterprise-accounts-billing.md
index 15e7d1079718..7e74130aadaa 100644
--- a/translations/ja-JP/data/reusables/enterprise-accounts/enterprise-accounts-billing.md
+++ b/translations/ja-JP/data/reusables/enterprise-accounts/enterprise-accounts-billing.md
@@ -1 +1 @@
-現在、Enterpriseアカウントはと、請求書での支払いをしている{% data variables.product.prodname_ghe_cloud %}と{% data variables.product.prodname_ghe_server %}のお客様が利用できます。 Enterpriseアカウントに接続されているすべてのOrganizationと{% data variables.product.prodname_ghe_server %}のインスタンスの請求は、1つの請求書に集約されます。 {% data variables.product.prodname_ghe_cloud %}サブスクリプションの管理に関する詳しい情報については、「[Enterpriseアカウントのサブスクリプションと利用状況の表示](/articles/viewing-the-subscription-and-usage-for-your-enterprise-account)」を参照してください。 For more information about managing your {% data variables.product.prodname_ghe_server %} billing settings, see "[Managing billing for your enterprise](/admin/overview/managing-billing-for-your-enterprise)."
+現在、Enterpriseアカウントはと、請求書での支払いをしている{% data variables.product.prodname_ghe_cloud %}と{% data variables.product.prodname_ghe_server %}のお客様が利用できます。 Enterpriseアカウントに接続されているすべてのOrganizationと{% data variables.product.prodname_ghe_server %}のインスタンスの請求は、1つの請求書に集約されます。
diff --git a/translations/ja-JP/data/reusables/enterprise_installation/hardware-considerations-all-platforms.md b/translations/ja-JP/data/reusables/enterprise_installation/hardware-considerations-all-platforms.md
index a08133cd7a47..756b5c40e8d0 100644
--- a/translations/ja-JP/data/reusables/enterprise_installation/hardware-considerations-all-platforms.md
+++ b/translations/ja-JP/data/reusables/enterprise_installation/hardware-considerations-all-platforms.md
@@ -1,4 +1,5 @@
- [Minimum requirements](#minimum-requirements)
+- [Beta features in {% data variables.product.prodname_ghe_server %} 2.22](#beta-features-in-github-enterprise-server-222)
- [ストレージ](#storage)
- [CPU and memory](#cpu-and-memory)
@@ -6,23 +7,18 @@
We recommend different hardware configurations depending on the number of user licenses for {% data variables.product.product_location %}. If you provision more resources than the minimum requirements, your instance will perform and scale better.
-{% data reusables.enterprise_installation.hardware-rec-table %} For more information about adjusting resources for an existing instance, see "[Increasing storage capacity](/enterprise/admin/installation/increasing-storage-capacity)" and "[Increasing CPU or memory resources](/enterprise/admin/installation/increasing-cpu-or-memory-resources)."
-
-{% if currentVersion == "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
-
-If you enable the beta for {% data variables.product.prodname_actions %} on your instance, we recommend planning for additional capacity.
+{% data reusables.enterprise_installation.hardware-rec-table %}{% if currentVersion == "enterprise-server@2.22" or currentVersion == "github-ae@latest" %} If you enable the beta for {% data variables.product.prodname_actions %}, review the following requirements and recommendations.
- You must configure at least one runner for {% data variables.product.prodname_actions %} workflows. 詳しい情報については「[セルフホストランナーについて](/actions/hosting-your-own-runners/about-self-hosted-runners)」を参照してください。
- You must configure external blob storage. 詳しい情報については、「[{% data variables.product.prodname_actions %} の有効化とストレージの設定](/enterprise/admin/github-actions/enabling-github-actions-and-configuring-storage)」をご覧ください。
-
-The additional CPU and memory resources you need to provision for your instance depend on the number of workflows your users run concurrently, and the overall levels of activity for users, automations, and integrations.
-
-| Maximum jobs per minute | vCPUs | メモリ |
-|:----------------------- | -----:| -------:|
-| Light testing | 4 | 30.5 GB |
-| 25 | 8 | 61 GB |
-| 35 | 16 | 122 GB |
-| 100 | 32 | 244 GB |
+- You may need to configure additional CPU and memory resources. The additional resources you need to provision for {% data variables.product.prodname_actions %} depend on the number of workflows your users run concurrently, and the overall levels of activity for users, automations, and integrations.
+
+ | Maximum jobs per minute | Additional vCPUs | Additional memory |
+ |:----------------------- | ----------------:| -----------------:|
+ | Light testing | 4 | 30.5 GB |
+ | 25 | 8 | 61 GB |
+ | 35 | 16 | 122 GB |
+ | 100 | 32 | 244 GB |
{% endif %}
diff --git a/translations/ja-JP/data/reusables/enterprise_installation/hardware-rec-table.md b/translations/ja-JP/data/reusables/enterprise_installation/hardware-rec-table.md
index 5b2ea6abde6e..df1cd374c411 100644
--- a/translations/ja-JP/data/reusables/enterprise_installation/hardware-rec-table.md
+++ b/translations/ja-JP/data/reusables/enterprise_installation/hardware-rec-table.md
@@ -1,6 +1,12 @@
{% if currentVersion == "enterprise-server@2.22" %}
-Minimum requirements for an instance with beta features enabled are **bold** in the following table. For more information, see "[Beta features in {% data variables.product.prodname_ghe_server %} 2.22](#beta-features-in-github-enterprise-server-222)." |{% endif %}
+{% note %}
+
+**Note**: If you enable beta features for {% data variables.product.prodname_ghe_server %} 2.22, your instance requires additional hardware resources. Minimum requirements for an instance with beta features enabled are **bold** in the following table. For more information about the features in beta, see "[Beta features in {% data variables.product.prodname_ghe_server %} 2.22](#beta-features-in-github-enterprise-server-222)."
+
+{% endnote %}
+|
+{% endif %}
| ユーザライセンス | vCPUs | メモリ | アタッチされたストレージ | ルートストレージ |
|:---------------------- | --------------------------------------------------------------------------------------------------------------------------------------:| ----------------------------------------------------------------------------------------------------------------------------------------------:| ----------------------------------------------------------------------------------------------------------------------------------------------:| --------:|
| トライアル、デモ、あるいは10人の軽量ユーザ | 2{% if currentVersion == "enterprise-server@2.22" %}
or [**4**](#beta-features-in-github-enterprise-server-222){% endif %} | 16 GB{% if currentVersion == "enterprise-server@2.22" %}
or [**32 GB**](#beta-features-in-github-enterprise-server-222){% endif %} | 100 GB{% if currentVersion == "enterprise-server@2.22" %}
or [**150 GB**](#beta-features-in-github-enterprise-server-222){% endif %} | 200 GB |
@@ -9,8 +15,14 @@ Minimum requirements for an instance with beta features enabled are **bold** in
| 5000-8000 | 12{% if currentVersion == "enterprise-server@2.22" %}
or [**16**](#beta-features-in-github-enterprise-server-222){% endif %} | 96 GB | 750 GB | 200 GB |
| 8000-10000+ | 16{% if currentVersion == "enterprise-server@2.22" %}
or [**20**](#beta-features-in-github-enterprise-server-222){% endif %} | 128 GB{% if currentVersion == "enterprise-server@2.22" %}
or [**160 GB**](#beta-features-in-github-enterprise-server-222){% endif %} | 1000 GB | 200 GB |
+For more information about adjusting resources for an existing instance, see "[Increasing storage capacity](/enterprise/admin/installation/increasing-storage-capacity)" and "[Increasing CPU or memory resources](/enterprise/admin/installation/increasing-cpu-or-memory-resources)."
+
{% if currentVersion == "enterprise-server@2.22" %}
#### Beta features in {% data variables.product.prodname_ghe_server %} 2.22
-If you enable beta features in {% data variables.product.prodname_ghe_server %} 2.22, your instance requires additional hardware resources. For more information about the beta features, see the [release notes for the 2.22 series](https://enterprise.github.com/releases/series/2.22) on the {% data variables.product.prodname_enterprise %} website.{% endif %}
+You can sign up for beta features available in {% data variables.product.prodname_ghe_server %} 2.22 such as {% data variables.product.prodname_actions %}, {% data variables.product.prodname_registry %}, and {% data variables.product.prodname_code_scanning %}. For more information, see the [release notes for the 2.22 series](https://enterprise.github.com/releases/series/2.22#release-2.22.0) on the {% data variables.product.prodname_enterprise %} website.
+
+If you enable beta features for {% data variables.product.prodname_ghe_server %} 2.22, your instance requires additional hardware resources. For more information, see "[Minimum requirements](#minimum-requirements)".
+
+{% endif %}
diff --git a/translations/ja-JP/data/reusables/enterprise_installation/image-urls-viewable-warning.md b/translations/ja-JP/data/reusables/enterprise_installation/image-urls-viewable-warning.md
index 189d674e32aa..2a26ad6d64f7 100644
--- a/translations/ja-JP/data/reusables/enterprise_installation/image-urls-viewable-warning.md
+++ b/translations/ja-JP/data/reusables/enterprise_installation/image-urls-viewable-warning.md
@@ -1,5 +1,5 @@
{% warning %}
-**警告:**Pull Request や Issue へのコメントに画像添付を追加した場合、その Pull Request がプライベートリポジトリのものであっても、またはプライベートモードが有効化されている場合であっても、匿名化した画像 URL は認証なしに誰もが見ることができます。 機密の画像をプライベートにしておきたい場合は、それらを認証が必要なプライベートなネットワークあるいはサーバーから提供するようにしてください。
+**Warning:** If you add an image attachment to a pull request or issue comment, anyone can view the anonymized image URL without authentication{% if enterpriseServerVersions contains currentVersion %}, even if the pull request is in a private repository, or if private mode is enabled. To prevent unauthorized access to the images, ensure that you restrict network access to the systems that serve the images, including {% data variables.product.product_location %}{% endif %}.{% if currentVersion == "github-ae@latest" %} To prevent unauthorized access to image URLs on {% data variables.product.product_name %}, consider restricting network traffic to your enterprise. For more information, see "[Restricting network traffic to your enterprise](/admin/configuration/restricting-network-traffic-to-your-enterprise)."{% endif %}
{% endwarning %}
diff --git a/translations/ja-JP/data/reusables/gated-features/pages.md b/translations/ja-JP/data/reusables/gated-features/pages.md
index e24925cc9877..31caf392d2d3 100644
--- a/translations/ja-JP/data/reusables/gated-features/pages.md
+++ b/translations/ja-JP/data/reusables/gated-features/pages.md
@@ -1 +1 @@
-{% data variables.product.prodname_pages %} is available in public repositories with {% data variables.product.prodname_free_user %} and {% data variables.product.prodname_free_team %} for organizations, and in public and private repositories with {% data variables.product.prodname_pro %}, {% data variables.product.prodname_team %}, {% data variables.product.prodname_ghe_cloud %},{% if currentVersion == "github-ae@latest" %} {% data variables.product.prodname_ghe_managed %},{% endif %} and {% data variables.product.prodname_ghe_server %}. {% if currentVersion == "free-pro-team@latest" %}{% data reusables.gated-features.more-info %}{% endif %}
+{% if currentVersion == "github-ae@latest" %}{% data variables.product.prodname_pages %} is available in internal and private repositories with {% data variables.product.prodname_ghe_managed %}. {% endif %}{% data variables.product.prodname_pages %} is available in public repositories with {% data variables.product.prodname_free_user %} and {% data variables.product.prodname_free_team %} for organizations, and in public and private repositories with {% data variables.product.prodname_pro %}, {% data variables.product.prodname_team %}, {% data variables.product.prodname_ghe_cloud %}, and {% data variables.product.prodname_ghe_server %}. {% if currentVersion == "free-pro-team@latest" %}{% data reusables.gated-features.more-info %}{% endif %}
diff --git a/translations/ja-JP/data/reusables/gated-features/wikis.md b/translations/ja-JP/data/reusables/gated-features/wikis.md
index 61f560896e02..d7611dc3638c 100644
--- a/translations/ja-JP/data/reusables/gated-features/wikis.md
+++ b/translations/ja-JP/data/reusables/gated-features/wikis.md
@@ -1 +1 @@
-Wikis are available in public repositories with {% data variables.product.prodname_free_user %} and {% data variables.product.prodname_free_team %} for organizations, and in public and private repositories with {% data variables.product.prodname_pro %}, {% data variables.product.prodname_team %}, {% data variables.product.prodname_ghe_cloud %},{% if currentVersion == "github-ae@latest" %} {% data variables.product.prodname_ghe_managed %},{% endif %} and {% data variables.product.prodname_ghe_server %}. {% if currentVersion == "free-pro-team@latest" %}{% data reusables.gated-features.more-info %}{% endif %}
+{% if currentVersion == "github-ae@latest" %}Wikis are available in internal and private repositories with {% data variables.product.prodname_ghe_managed %}.{% endif %} Wikis are available in public repositories with {% data variables.product.prodname_free_user %} and {% data variables.product.prodname_free_team %} for organizations, and in public and private repositories with {% data variables.product.prodname_pro %}, {% data variables.product.prodname_team %}, {% data variables.product.prodname_ghe_cloud %} and {% data variables.product.prodname_ghe_server %}. {% if currentVersion == "free-pro-team@latest" %}{% data reusables.gated-features.more-info %}{% endif %}
diff --git a/translations/ja-JP/data/reusables/github-actions/self-hosted-runner-configure-runner-group-access.md b/translations/ja-JP/data/reusables/github-actions/self-hosted-runner-configure-runner-group-access.md
index 0cd319c4ae2c..9ac93498fa1e 100644
--- a/translations/ja-JP/data/reusables/github-actions/self-hosted-runner-configure-runner-group-access.md
+++ b/translations/ja-JP/data/reusables/github-actions/self-hosted-runner-configure-runner-group-access.md
@@ -1,2 +1,12 @@
-1. In the **Self-hosted runners** section of the settings page, click {% octicon "kebab-horizontal" aria-label="The horizontal kebab icon" %} next to the runner group you'd like to configure, then click **Edit name and [organization|repository] access**. 
-1. Select a new policy from the dropdown list, or modify the runner group name.
+1. In the **Self-hosted runners** section of the settings page, click {% octicon "kebab-horizontal" aria-label="The horizontal kebab icon" %} next to the runner group you'd like to configure, then click **Edit name and [organization|repository] access**.
+ 
+1. Modify your policy options, or change the runner group name.
+
+ {% warning %}
+
+ **Warning**
+ {% indented_data_reference site.data.reusables.github-actions.self-hosted-runner-security spaces=3 %}
+ For more information, see "[About self-hosted runners](/actions/hosting-your-own-runners/about-self-hosted-runners#self-hosted-runner-security-with-public-repositories)."
+
+ {% endwarning %}
+
diff --git a/translations/ja-JP/data/reusables/notifications-v2/custom-notifications-beta.md b/translations/ja-JP/data/reusables/notifications-v2/custom-notifications-beta.md
new file mode 100644
index 000000000000..dbcee80cfe1d
--- /dev/null
+++ b/translations/ja-JP/data/reusables/notifications-v2/custom-notifications-beta.md
@@ -0,0 +1,9 @@
+{% if currentVersion == "free-pro-team@latest" %}
+
+{% note %}
+
+**Note:** Custom notifications are currently in beta and subject to change.
+
+{% endnote %}
+
+{% endif %}
diff --git a/translations/ja-JP/data/reusables/notifications/outbound_email_tip.md b/translations/ja-JP/data/reusables/notifications/outbound_email_tip.md
index d4dc201cc038..ab27e597f5fe 100644
--- a/translations/ja-JP/data/reusables/notifications/outbound_email_tip.md
+++ b/translations/ja-JP/data/reusables/notifications/outbound_email_tip.md
@@ -1,7 +1,9 @@
-{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
- {% tip %}
+{% if enterpriseServerVersions contains currentVersion %}
- {% data variables.product.product_location %}上でアウトバウンドメールのサポートが有効化されている場合にのみ、メール通知を受信することになります。 詳しい情報については、サイト管理者にお問い合わせください。
+{% note %}
+
+**Note**: You'll only receive email notifications if outbound email support is enabled on {% data variables.product.product_location %}. 詳しい情報については、サイト管理者にお問い合わせください。
+
+{% endnote %}
- {% endtip %}
{% endif %}
diff --git a/translations/ja-JP/data/reusables/notifications/shared_state.md b/translations/ja-JP/data/reusables/notifications/shared_state.md
index b85150b448d3..7ab93ffea2cd 100644
--- a/translations/ja-JP/data/reusables/notifications/shared_state.md
+++ b/translations/ja-JP/data/reusables/notifications/shared_state.md
@@ -1,5 +1,5 @@
{% tip %}
-**Tip:** Web通知とメール通知の両方を受信する場合、通知の既読あるいは未読状態を自動的に同期して、対応するメール通知を読んだら自動的にWeb通知が既読としてマークされるようにできます。 To enable this sync, your email client must be able to view images from {% if currentVersion == "free-pro-team@latest" %}'`notifications@github.com`'{% else %}'the no-reply email address configured by your site administrator'{% endif %}.
+**Tip:** Web通知とメール通知の両方を受信する場合、通知の既読あるいは未読状態を自動的に同期して、対応するメール通知を読んだら自動的にWeb通知が既読としてマークされるようにできます。 To enable this sync, your email client must be able to view images from {% if currentVersion == "free-pro-team@latest" %}`notifications@github.com`{% else %}the `no-reply` email address {% if currentVersion == "github-ae@latest" %}for your {% data variables.product.product_name %} hostname{% elsif enterpriseServerVersions contains currentVersion %}for {% data variables.product.product_location %}, which your site administrator configures{% endif %}{% endif %}.
{% endtip %}
diff --git a/translations/ja-JP/data/reusables/profile/profile-readme.md b/translations/ja-JP/data/reusables/profile/profile-readme.md
index a19a3d4a30d3..7350ed57bd30 100644
--- a/translations/ja-JP/data/reusables/profile/profile-readme.md
+++ b/translations/ja-JP/data/reusables/profile/profile-readme.md
@@ -1 +1 @@
-If you add a README file to the root of a public repository with the same name as your username, that README will automatically appear on your profile page. You can edit your profile README with GitHub Flavored Markdown to create a personalized section on your profile.
+If you add a README file to the root of a public repository with the same name as your username, that README will automatically appear on your profile page. You can edit your profile README with {% data variables.product.company_short %} Flavored Markdown to create a personalized section on your profile. For more information, see "[Managing your profile README](/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme)."
diff --git a/translations/ja-JP/data/reusables/repositories/administrators-can-disable-issues.md b/translations/ja-JP/data/reusables/repositories/administrators-can-disable-issues.md
new file mode 100644
index 000000000000..c21943def063
--- /dev/null
+++ b/translations/ja-JP/data/reusables/repositories/administrators-can-disable-issues.md
@@ -0,0 +1 @@
+Repository administrators can disable issues for a repository. 詳細は「[Issue を無効化する](/github/managing-your-work-on-github/disabling-issues)」を参照してください。
diff --git a/translations/ja-JP/data/reusables/repositories/desktop-fork.md b/translations/ja-JP/data/reusables/repositories/desktop-fork.md
index 64162611916c..87352ea86dd8 100644
--- a/translations/ja-JP/data/reusables/repositories/desktop-fork.md
+++ b/translations/ja-JP/data/reusables/repositories/desktop-fork.md
@@ -1 +1 @@
-{% data variables.product.prodname_desktop %}を使ってリポジトリのフォークをすることができます。 詳しい情報については「[{% data variables.product.prodname_desktop %}からのリポジトリのクローンとフォーク](/desktop/contributing-to-projects/cloning-and-forking-repositories-from-github-desktop)」を参照してください。
+{% data variables.product.prodname_desktop %}を使ってリポジトリのフォークをすることができます。 For more information, see "[Cloning and forking repositories from {% data variables.product.prodname_desktop %}](/desktop/contributing-to-projects/cloning-and-forking-repositories-from-github-desktop)."
diff --git a/translations/ja-JP/data/reusables/repositories/you-can-fork.md b/translations/ja-JP/data/reusables/repositories/you-can-fork.md
index 6ba41cc13ba7..691c670483da 100644
--- a/translations/ja-JP/data/reusables/repositories/you-can-fork.md
+++ b/translations/ja-JP/data/reusables/repositories/you-can-fork.md
@@ -1,3 +1,7 @@
-任意のパブリックなリポジトリを、自分のユーザアカウントあるいはリポジトリの作成権限を持っている任意のOrganization にフォークできます。 詳細は「[Organization の権限レベル](/articles/permission-levels-for-an-organization)」を参照してください。
+{% if currentVersion == "github-ae@latest" %}If the policies for your enterprise permit forking internal and private repositories, you{% else %}You{% endif %} can fork a repository to your user account or any organization where you have repository creation permissions. 詳細は「[Organization の権限レベル](/articles/permission-levels-for-an-organization)」を参照してください。
-アクセス権を持っている任意のプライベートリポジトリを、自分のユーザアカウント及びリポジトリの作成権限を持っている{% data variables.product.prodname_team %}もしくは{% data variables.product.prodname_enterprise %}上の任意のOrganizationにフォークできます。 You cannot fork a private repository to an organization using {% data variables.product.prodname_free_team %}.{% if currentVersion == "free-pro-team@latest" %} For more information, see "[GitHub's products](/articles/githubs-products)."{% endif %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+If you have access to a private repository and the owner permits forking, you can fork the repository to your user account or any organization on {% if currentVersion == "free-pro-team@latest"%}{% data variables.product.prodname_team %}{% else %}{% data variables.product.product_location %}{% endif %} where you have repository creation permissions. {% if currentVersion == "free-pro-team@latest" %}You cannot fork a private repository to an organization using {% data variables.product.prodname_free_team %}. For more information, see "[GitHub's products](/articles/githubs-products)."{% endif %}
+
+{% endif %}
\ No newline at end of file
diff --git a/translations/ja-JP/data/reusables/search/required_login.md b/translations/ja-JP/data/reusables/search/required_login.md
index f820ba18f235..6d8598a94a38 100644
--- a/translations/ja-JP/data/reusables/search/required_login.md
+++ b/translations/ja-JP/data/reusables/search/required_login.md
@@ -1 +1 @@
-すべてのパブリックリポジトリにわたってコードを検索するには、サインインしなければなりません。
+You must be signed into a user account on {% data variables.product.product_name %} to search for code across all public repositories.
diff --git a/translations/ja-JP/data/reusables/search/syntax_tips.md b/translations/ja-JP/data/reusables/search/syntax_tips.md
index b3f0c1d8599e..8ee338dcf3b0 100644
--- a/translations/ja-JP/data/reusables/search/syntax_tips.md
+++ b/translations/ja-JP/data/reusables/search/syntax_tips.md
@@ -1,7 +1,7 @@
{% tip %}
**Tips:**{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
- - この記事には、{% data variables.product.prodname_dotcom %}.com のウェブサイトでの検索例が含まれています。ですが、同じ検索フィルターを {% data variables.product.product_location %} で使えます。{% endif %}
+ - This article contains links to example searches on the {% data variables.product.prodname_dotcom_the_website %} website, but you can use the same search filters with {% data variables.product.product_name %}. In the linked example searches, replace `github.com` with the hostname for {% data variables.product.product_location %}.{% endif %}
- 検索結果を改良する検索修飾子を追加できる検索構文のリストについては、「[検索構文を理解する](/articles/understanding-the-search-syntax)」を参照してください。
- 複数単語の検索用語は引用符で囲みます。 たとえば "In progress" というラベルを持つ Issue を検索したい場合は、`label:"in progress"` とします。 検索では、大文字と小文字は区別されません。
diff --git a/translations/ja-JP/data/reusables/search/you-can-search-globally.md b/translations/ja-JP/data/reusables/search/you-can-search-globally.md
new file mode 100644
index 000000000000..abb221f6ba61
--- /dev/null
+++ b/translations/ja-JP/data/reusables/search/you-can-search-globally.md
@@ -0,0 +1 @@
+{% data variables.product.product_name %}全体にわたってグローバルに検索できます。あるいは、検索を特定のリポジトリや Organization に絞ることもできます。
diff --git a/translations/ja-JP/data/reusables/ssh/add-ssh-key-to-ssh-agent-commandline.md b/translations/ja-JP/data/reusables/ssh/add-ssh-key-to-ssh-agent-commandline.md
index af4760bd1725..415f86736de3 100644
--- a/translations/ja-JP/data/reusables/ssh/add-ssh-key-to-ssh-agent-commandline.md
+++ b/translations/ja-JP/data/reusables/ssh/add-ssh-key-to-ssh-agent-commandline.md
@@ -1,3 +1,3 @@
```shell
-$ ssh-add ~/.ssh/id_rsa
+$ ssh-add ~/.ssh/id_ed25519
```
diff --git a/translations/ja-JP/data/reusables/ssh/add-ssh-key-to-ssh-agent.md b/translations/ja-JP/data/reusables/ssh/add-ssh-key-to-ssh-agent.md
index 3e32f9ee2e32..fb1ca5ec3428 100644
--- a/translations/ja-JP/data/reusables/ssh/add-ssh-key-to-ssh-agent.md
+++ b/translations/ja-JP/data/reusables/ssh/add-ssh-key-to-ssh-agent.md
@@ -1 +1 @@
-キーを別の名前で作成したか、別の名前を持つ既存のキーを追加しようとしている場合は、コマンド内の*id_rsa*を秘密鍵ファイルの名前で置き換えてください。
+If you created your key with a different name, or if you are adding an existing key that has a different name, replace *id_ed25519* in the command with the name of your private key file.
diff --git a/translations/ja-JP/data/variables/action_code_examples.yml b/translations/ja-JP/data/variables/action_code_examples.yml
index 24571dbda3a1..fcbcb9c899db 100644
--- a/translations/ja-JP/data/variables/action_code_examples.yml
+++ b/translations/ja-JP/data/variables/action_code_examples.yml
@@ -64,7 +64,7 @@
- publishing
-
title: GitHub Project Automation+
- description: Automate GitHub Project cards with any webhook event.
+ description: Automate GitHub Project cards with any webhook event
languages: JavaScript
href: alex-page/github-project-automation-plus
tags:
@@ -131,8 +131,7 @@
- publishing
-
title: Label your Pull Requests auto-magically (using committed files)
- description: >-
- Github action to label your pull requests auto-magically (using committed files)
+ description: Github action to label your pull requests auto-magically (using committed files)
languages: 'TypeScript, Dockerfile, JavaScript'
href: Decathlon/pull-request-labeler-action
tags:
@@ -147,3 +146,191 @@
tags:
- プルリクエスト
- labels
+-
+ title: Get a list of file changes with a PR/Push
+ description: This action gives you will get outputs of the files that have changed in your repository
+ languages: 'TypeScript, Shell, JavaScript'
+ href: trilom/file-changes-action
+ tags:
+ - ワークフロー
+ - リポジトリ
+-
+ title: Private actions in any workflow
+ description: Allows private GitHub Actions to be easily reused
+ languages: 'TypeScript, JavaScript, Shell'
+ href: InVisionApp/private-action-loader
+ tags:
+ - ワークフロー
+ - tools
+-
+ title: Label your issues using the issue's contents
+ description: A GitHub Action to automatically tag issues with labels and assignees
+ languages: 'JavaScript, TypeScript'
+ href: damccorm/tag-ur-it
+ tags:
+ - ワークフロー
+ - tools
+ - labels
+ - issues
+-
+ title: Rollback a GitHub Release
+ description: A GitHub Action to rollback or delete a release
+ languages: 'JavaScript'
+ href: author/action-rollback
+ tags:
+ - ワークフロー
+ - releases
+-
+ title: Lock closed issues and Pull Requests
+ description: GitHub Action that locks closed issues and pull requests after a period of inactivity
+ languages: 'JavaScript'
+ href: dessant/lock-threads
+ tags:
+ - issues
+ - プルリクエスト
+ - ワークフロー
+-
+ title: Get Commit Difference Count Between Two Branches
+ description: This GitHub Action compares two branches and gives you the commit count between them
+ languages: 'JavaScript, Shell'
+ href: jessicalostinspace/commit-difference-action
+ tags:
+ - コミット
+ - diff
+ - ワークフロー
+-
+ title: Generate Release Notes Based on Git References
+ description: GitHub Action to generate changelogs, and release notes
+ languages: 'JavaScript, Shell'
+ href: metcalfc/changelog-generator
+ tags:
+ - cicd
+ - release-notes
+ - ワークフロー
+ - 変更履歴
+-
+ title: Enforce Policies on GitHub Repositories and Commits
+ description: Policy enforcement for your pipelines
+ languages: 'Go, Makefile, Dockerfile, Shell'
+ href: talos-systems/conform
+ tags:
+ - docker
+ - build-automation
+ - ワークフロー
+-
+ title: Auto Label Issue Based
+ description: Automatically label an issue based on the issue description
+ languages: 'TypeScript, JavaScript, Dockerfile'
+ href: Renato66/auto-label
+ tags:
+ - labels
+ - ワークフロー
+ - automation
+-
+ title: Update Configured GitHub Actions to the Latest Versions
+ description: CLI tool to check whehter all your actions are up-to-date or not
+ languages: 'C#, Inno Setup, PowerSHell, Shell'
+ href: fabasoad/ghacu
+ tags:
+ - versions
+ - cli
+ - ワークフロー
+-
+ title: Create Issue Branch
+ description: GitHub Action that automates the creation of issue branches
+ languages: 'JavaScript, Shell'
+ href: robvanderleek/create-issue-branch
+ tags:
+ - probot
+ - issues
+ - labels
+-
+ title: Remove old artifacts
+ description: Customize artifact cleanup
+ languages: 'JavaScript, Shell'
+ href: c-hive/gha-remove-artifacts
+ tags:
+ - 成果物
+ - ワークフロー
+-
+ title: Sync Defined Files/Binaries to Wiki or External Repositories
+ description: GitHub Action to automatically sync changes to external repositories, like the wiki, for example
+ languages: 'Shell, Dockerfile'
+ href: kai-tub/external-repo-sync-action
+ tags:
+ - wiki
+ - sync
+ - ワークフロー
+-
+ title: Create/Update/Delete a GitHub Wiki page based on any file
+ description: Updates your GitHub wiki by using rsync, allowing for exclusion of files and directories and actual deletion of files
+ languages: 'Shell, Dockerfile'
+ href: Andrew-Chen-Wang/github-wiki-action
+ tags:
+ - wiki
+ - docker
+ - ワークフロー
+-
+ title: Prow GitHub Actions
+ description: Automation of policy enforcement, chat-ops, and automatic PR merging
+ languages: 'TypeScript, JavaScript'
+ href: jpmcb/prow-github-actions
+ tags:
+ - chat-ops
+ - prow
+ - ワークフロー
+-
+ title: Check GitHub Status in your Workflow
+ description: Check GitHub Status in your workflow
+ languages: 'TypeScript, JavaScript'
+ href: crazy-max/ghaction-github-status
+ tags:
+ - ステータス
+ - モニタリング
+ - ワークフロー
+-
+ title: Manage labels on GitHub as code
+ description: GitHub Action to manage labels (create/rename/update/delete)
+ languages: 'TypeScript, JavaScript'
+ href: crazy-max/ghaction-github-labeler
+ tags:
+ - labels
+ - ワークフロー
+ - automation
+-
+ title: Distribute funding in free and open source projects
+ description: Continuous Distribution of funding to project contributors and dependencies
+ languages: 'Python, Docerfile, Shell, Ruby'
+ href: protontypes/libreselery
+ tags:
+ - sponsors
+ - funding
+ - payment
+-
+ title: Herald rules for GitHub
+ description: Add reviewers, subscribers, labels and assignees to your PR
+ languages: 'TypeScript, JavaScript'
+ href: gagoar/use-herald-action
+ tags:
+ - reviewers
+ - labels
+ - assignees
+ - プルリクエスト
+-
+ title: Codeowner validator
+ description: Ensures the correctness of your GitHub CODEOWNERS file, supports public and private GitHub repositories and also GitHub Enterprise installations
+ languages: 'Go, Shell, Makefile, Docerfile'
+ href: mszostok/codeowners-validator
+ tags:
+ - codeowners
+ - validate
+ - ワークフロー
+-
+ title: Copybara Action
+ description: Move and transform code between repositories (ideal to maintain several repos from one monorepo)
+ languages: 'TypeScript, JavaScript, Shell'
+ href: olivr/copybara-action
+ tags:
+ - monorepo
+ - copybara
+ - ワークフロー
diff --git a/translations/ko-KR/content/actions/hosting-your-own-runners/managing-access-to-self-hosted-runners-using-groups.md b/translations/ko-KR/content/actions/hosting-your-own-runners/managing-access-to-self-hosted-runners-using-groups.md
index e0d1e320cdd1..b23291c04ac6 100644
--- a/translations/ko-KR/content/actions/hosting-your-own-runners/managing-access-to-self-hosted-runners-using-groups.md
+++ b/translations/ko-KR/content/actions/hosting-your-own-runners/managing-access-to-self-hosted-runners-using-groups.md
@@ -33,7 +33,7 @@ All organizations have a single default self-hosted runner group. Organizations
Self-hosted runners are automatically assigned to the default group when created, and can only be members of one group at a time. You can move a runner from the default group to any group you create.
-When creating a group, you must choose a policy that defines which repositories have access to the runner group. You can configure a runner group to be accessible to a specific list of repositories, all private repositories, or all repositories in the organization.
+When creating a group, you must choose a policy that defines which repositories have access to the runner group.
{% data reusables.organizations.navigate-to-org %}
{% data reusables.organizations.org_settings %}
@@ -41,9 +41,19 @@ When creating a group, you must choose a policy that defines which repositories
1. In the **Self-hosted runners** section, click **Add new**, and then **New group**.

-1. Enter a name for your runner group, and select an access policy from the **Repository access** dropdown list.
+1. Enter a name for your runner group, and assign a policy for repository access.
- 
+ {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %} You can configure a runner group to be accessible to a specific list of repositories, or to all repositories in the organization. By default, public repositories can't access runners in a runner group, but you can use the **Allow public repositories** option to override this.{% else if currentVersion == "enterprise-server@2.22"%}You can configure a runner group to be accessible to a specific list of repositories, all private repositories, or all repositories in the organization.{% endif %}
+
+ {% warning %}
+
+ **경고**
+ {% indented_data_reference site.data.reusables.github-actions.self-hosted-runner-security spaces=3 %}
+ For more information, see "[About self-hosted runners](/actions/hosting-your-own-runners/about-self-hosted-runners#self-hosted-runner-security-with-public-repositories)."
+
+ {% endwarning %}
+
+ 
1. Click **Save group** to create the group and apply the policy.
### Creating a self-hosted runner group for an enterprise
@@ -52,7 +62,7 @@ Enterprises can add their self-hosted runners to groups for access management. E
Self-hosted runners are automatically assigned to the default group when created, and can only be members of one group at a time. You can assign the runner to a specific group during the registration process, or you can later move the runner from the default group to a custom group.
-When creating a group, you must choose a policy that grants access to all organizations in the enterprise or choose specific organizations.
+When creating a group, you must choose a policy that defines which organizations have access to the runner group.
{% data reusables.enterprise-accounts.access-enterprise %}
{% data reusables.enterprise-accounts.policies-tab %}
@@ -61,7 +71,17 @@ When creating a group, you must choose a policy that grants access to all organi
1. Click **Add new**, and then **New group**.

-1. Enter a name for your runner group, and select an access policy from the **Organization access** dropdown list.
+1. Enter a name for your runner group, and assign a policy for organization access.
+
+ {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %} You can configure a runner group to be accessible to a specific list of organizations, or all organizations in the enterprise. By default, public repositories can't access runners in a runner group, but you can use the **Allow public repositories** option to override this.{% else if currentVersion == "enterprise-server@2.22"%}You can configure a runner group to be accessible to all organizations in the enterprise or choose specific organizations.{% endif %}
+
+ {% warning %}
+
+ **경고**
+ {% indented_data_reference site.data.reusables.github-actions.self-hosted-runner-security spaces=3 %}
+ For more information, see "[About self-hosted runners](/actions/hosting-your-own-runners/about-self-hosted-runners#self-hosted-runner-security-with-public-repositories)."
+
+ {% endwarning %}

1. Click **Save group** to create the group and apply the policy.
diff --git a/translations/ko-KR/content/actions/reference/events-that-trigger-workflows.md b/translations/ko-KR/content/actions/reference/events-that-trigger-workflows.md
index b73aa98a496a..067053a5eec3 100644
--- a/translations/ko-KR/content/actions/reference/events-that-trigger-workflows.md
+++ b/translations/ko-KR/content/actions/reference/events-that-trigger-workflows.md
@@ -572,6 +572,8 @@ on:
{% data reusables.developer-site.pull_request_forked_repos_link %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %}
+
#### `pull_request_target`
This event is similar to `pull_request`, except that it runs in the context of the base repository of the pull request, rather than in the merge commit. This means that you can more safely make your secrets available to the workflows triggered by the pull request, because only workflows defined in the commit on the base repository are run. For example, this event allows you to create workflows that label and comment on pull requests, based on the contents of the event payload.
@@ -589,6 +591,8 @@ on: pull_request_target
types: [assigned, opened, synchronize, reopened]
```
+{% endif %}
+
#### `푸시`
{% note %}
@@ -689,6 +693,8 @@ on:
types: [started]
```
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %}
+
#### `workflow_run`
{% data reusables.webhooks.workflow_run_desc %}
@@ -711,6 +717,8 @@ on:
- requested
```
+{% endif %}
+
### Triggering new workflows using a personal access token
{% data reusables.github-actions.actions-do-not-trigger-workflows %} For more information, see "[Authenticating with the GITHUB_TOKEN](/actions/configuring-and-managing-workflows/authenticating-with-the-github_token)."
diff --git a/translations/ko-KR/content/actions/reference/workflow-syntax-for-github-actions.md b/translations/ko-KR/content/actions/reference/workflow-syntax-for-github-actions.md
index 636aa0364e54..1a163d656951 100644
--- a/translations/ko-KR/content/actions/reference/workflow-syntax-for-github-actions.md
+++ b/translations/ko-KR/content/actions/reference/workflow-syntax-for-github-actions.md
@@ -876,6 +876,37 @@ strategy:
{% endnote %}
+##### Using environment variables in a matrix
+
+You can add custom environment variables for each test combination by using `include` with `env`. You can then refer to the custom environment variables in a later step.
+
+In this example, the matrix entries for `node-version` are each configured to use different values for the `site` and `datacenter` environment variables. The `Echo site details` step then uses {% raw %}`env: ${{ matrix.env }}`{% endraw %} to refer to the custom variables:
+
+{% raw %}
+```yaml
+name: Node.js CI
+on: [push]
+jobs:
+ build:
+ runs-on: ubuntu-latest
+ strategy:
+ matrix:
+ include:
+ - node-version: 10.x
+ site: "prod"
+ datacenter: "site-a"
+ - node-version: 12.x
+ site: "dev"
+ datacenter: "site-b"
+ steps:
+ - name: Echo site details
+ env:
+ SITE: ${{ matrix.site }}
+ DATACENTER: ${{ matrix.datacenter }}
+ run: echo $SITE $DATACENTER
+```
+{% endraw %}
+
### **`jobs..strategy.fail-fast`**
When set to `true`, {% data variables.product.prodname_dotcom %} cancels all in-progress jobs if any `matrix` job fails. Default: `true`
diff --git a/translations/ko-KR/content/admin/configuration/command-line-utilities.md b/translations/ko-KR/content/admin/configuration/command-line-utilities.md
index f2ea220519b9..c9e22e1bdb64 100644
--- a/translations/ko-KR/content/admin/configuration/command-line-utilities.md
+++ b/translations/ko-KR/content/admin/configuration/command-line-utilities.md
@@ -84,7 +84,7 @@ Allows you to find the uuid of your node in `cluster.conf`.
Allows you to exempt a list of users from API rate limits. For more information, see "[Resources in the REST API](/rest/overview/resources-in-the-rest-api#rate-limiting)."
``` shell
-$ ghe-config app.github.rate_limiting_exempt_users "hubot github-actions"
+$ ghe-config app.github.rate-limiting-exempt-users "hubot github-actions"
# Exempts the users hubot and github-actions from rate limits
```
{% endif %}
diff --git a/translations/ko-KR/content/admin/enterprise-management/configuring-high-availability-replication-for-a-cluster.md b/translations/ko-KR/content/admin/enterprise-management/configuring-high-availability-replication-for-a-cluster.md
index 1cf5af72dba8..e244198a381e 100644
--- a/translations/ko-KR/content/admin/enterprise-management/configuring-high-availability-replication-for-a-cluster.md
+++ b/translations/ko-KR/content/admin/enterprise-management/configuring-high-availability-replication-for-a-cluster.md
@@ -57,32 +57,36 @@ Before you define a secondary datacenter for your passive nodes, ensure that you
mysql-master = HOSTNAME
redis-master = HOSTNAME
primary-datacenter = default
- ```
+ ```
- Optionally, change the name of the primary datacenter to something more descriptive or accurate by editing the value of `primary-datacenter`.
4. {% data reusables.enterprise_clustering.configuration-file-heading %} Under each node's heading, add a new key-value pair to assign the node to a datacenter. Use the same value as `primary-datacenter` from step 3 above. For example, if you want to use the default name (`default`), add the following key-value pair to the section for each node.
- datacenter = default
+ ```
+ datacenter = default
+ ```
When you're done, the section for each node in the cluster configuration file should look like the following example. {% data reusables.enterprise_clustering.key-value-pair-order-irrelevant %}
- ```shell
- [cluster "HOSTNAME"]
- datacenter = default
- hostname = HOSTNAME
- ipv4 = IP ADDRESS
+ ```shell
+ [cluster "HOSTNAME"]
+ datacenter = default
+ hostname = HOSTNAME
+ ipv4 = IP ADDRESS
+ ...
...
- ...
- ```
+ ```
- {% note %}
+ {% note %}
- **Note**: If you changed the name of the primary datacenter in step 3, find the `consul-datacenter` key-value pair in the section for each node and change the value to the renamed primary datacenter. For example, if you named the primary datacenter `primary`, use the following key-value pair for each node.
+ **Note**: If you changed the name of the primary datacenter in step 3, find the `consul-datacenter` key-value pair in the section for each node and change the value to the renamed primary datacenter. For example, if you named the primary datacenter `primary`, use the following key-value pair for each node.
- consul-datacenter = primary
+ ```
+ consul-datacenter = primary
+ ```
- {% endnote %}
+ {% endnote %}
{% data reusables.enterprise_clustering.apply-configuration %}
@@ -103,31 +107,37 @@ For an example configuration, see "[Example configuration](#example-configuratio
1. For each node in your cluster, provision a matching virtual machine with identical specifications, running the same version of {% data variables.product.prodname_ghe_server %}. Note the IPv4 address and hostname for each new cluster node. For more information, see "[Prerequisites](#prerequisites)."
- {% note %}
+ {% note %}
- **Note**: If you're reconfiguring high availability after a failover, you can use the old nodes from the primary datacenter instead.
+ **Note**: If you're reconfiguring high availability after a failover, you can use the old nodes from the primary datacenter instead.
- {% endnote %}
+ {% endnote %}
{% data reusables.enterprise_clustering.ssh-to-a-node %}
3. Back up your existing cluster configuration.
-
- cp /data/user/common/cluster.conf ~/$(date +%Y-%m-%d)-cluster.conf.backup
+
+ ```
+ cp /data/user/common/cluster.conf ~/$(date +%Y-%m-%d)-cluster.conf.backup
+ ```
4. Create a copy of your existing cluster configuration file in a temporary location, like _/home/admin/cluster-passive.conf_. Delete unique key-value pairs for IP addresses (`ipv*`), UUIDs (`uuid`), and public keys for WireGuard (`wireguard-pubkey`).
-
- grep -Ev "(?:|ipv|uuid|vpn|wireguard\-pubkey)" /data/user/common/cluster.conf > ~/cluster-passive.conf
+
+ ```
+ grep -Ev "(?:|ipv|uuid|vpn|wireguard\-pubkey)" /data/user/common/cluster.conf > ~/cluster-passive.conf
+ ```
5. Remove the `[cluster]` section from the temporary cluster configuration file that you copied in the previous step.
-
- git config -f ~/cluster-passive.conf --remove-section cluster
+
+ ```
+ git config -f ~/cluster-passive.conf --remove-section cluster
+ ```
6. Decide on a name for the secondary datacenter where you provisioned your passive nodes, then update the temporary cluster configuration file with the new datacenter name. Replace `SECONDARY` with the name you choose.
```shell
- sed -i 's/datacenter = default/datacenter = SECONDARY/g' ~/cluster-passive.conf
- ```
+ sed -i 's/datacenter = default/datacenter = SECONDARY/g' ~/cluster-passive.conf
+ ```
7. Decide on a pattern for the passive nodes' hostnames.
@@ -140,7 +150,7 @@ For an example configuration, see "[Example configuration](#example-configuratio
8. Open the temporary cluster configuration file from step 3 in a text editor. For example, you can use Vim.
```shell
- sudo vim ~/cluster-passive.conf
+ sudo vim ~/cluster-passive.conf
```
9. In each section within the temporary cluster configuration file, update the node's configuration. {% data reusables.enterprise_clustering.configuration-file-heading %}
@@ -150,37 +160,37 @@ For an example configuration, see "[Example configuration](#example-configuratio
- Add a new key-value pair, `replica = enabled`.
```shell
- [cluster "NEW PASSIVE NODE HOSTNAME"]
+ [cluster "NEW PASSIVE NODE HOSTNAME"]
+ ...
+ hostname = NEW PASSIVE NODE HOSTNAME
+ ipv4 = NEW PASSIVE NODE IPV4 ADDRESS
+ replica = enabled
+ ...
...
- hostname = NEW PASSIVE NODE HOSTNAME
- ipv4 = NEW PASSIVE NODE IPV4 ADDRESS
- replica = enabled
- ...
- ...
```
10. Append the contents of the temporary cluster configuration file that you created in step 4 to the active configuration file.
```shell
- cat ~/cluster-passive.conf >> /data/user/common/cluster.conf
- ```
+ cat ~/cluster-passive.conf >> /data/user/common/cluster.conf
+ ```
11. Designate the primary MySQL and Redis nodes in the secondary datacenter. Replace `REPLICA MYSQL PRIMARY HOSTNAME` and `REPLICA REDIS PRIMARY HOSTNAME` with the hostnames of the passives node that you provisioned to match your existing MySQL and Redis primaries.
```shell
- git config -f /data/user/common/cluster.conf cluster.mysql-master-replica REPLICA MYSQL PRIMARY HOSTNAME
- git config -f /data/user/common/cluster.conf cluster.redis-master-replica REPLICA REDIS PRIMARY HOSTNAME
- ```
+ git config -f /data/user/common/cluster.conf cluster.mysql-master-replica REPLICA MYSQL PRIMARY HOSTNAME
+ git config -f /data/user/common/cluster.conf cluster.redis-master-replica REPLICA REDIS PRIMARY HOSTNAME
+ ```
12. Enable MySQL to fail over automatically when you fail over to the passive replica nodes.
```shell
- git config -f /data/user/common/cluster.conf cluster.mysql-auto-failover true
+ git config -f /data/user/common/cluster.conf cluster.mysql-auto-failover true
```
- {% warning %}
+ {% warning %}
- **Warning**: Review your cluster configuration file before proceeding.
+ **Warning**: Review your cluster configuration file before proceeding.
- In the top-level `[cluster]` section, ensure that the values for `mysql-master-replica` and `redis-master-replica` are the correct hostnames for the passive nodes in the secondary datacenter that will serve as the MySQL and Redis primaries after a failover.
- In each section for an active node named `[cluster "ACTIVE NODE HOSTNAME"]`, double-check the following key-value pairs.
@@ -194,9 +204,9 @@ For an example configuration, see "[Example configuration](#example-configuratio
- `replica` should be configured as `enabled`.
- Take the opportunity to remove sections for offline nodes that are no longer in use.
- To review an example configuration, see "[Example configuration](#example-configuration)."
+ To review an example configuration, see "[Example configuration](#example-configuration)."
- {% endwarning %}
+ {% endwarning %}
13. Initialize the new cluster configuration. {% data reusables.enterprise.use-a-multiplexer %}
@@ -207,7 +217,7 @@ For an example configuration, see "[Example configuration](#example-configuratio
14. After the initialization finishes, {% data variables.product.prodname_ghe_server %} displays the following message.
```shell
- Finished cluster initialization
+ Finished cluster initialization
```
{% data reusables.enterprise_clustering.apply-configuration %}
@@ -293,20 +303,28 @@ Initial replication between the active and passive nodes in your cluster takes t
You can monitor the progress on any node in the cluster, using command-line tools available via the {% data variables.product.prodname_ghe_server %} administrative shell. For more information about the administrative shell, see "[Accessing the administrative shell (SSH)](/enterprise/admin/configuration/accessing-the-administrative-shell-ssh)."
- Monitor replication of databases:
-
- /usr/local/share/enterprise/ghe-cluster-status-mysql
+
+ ```
+ /usr/local/share/enterprise/ghe-cluster-status-mysql
+ ```
- Monitor replication of repository and Gist data:
-
- ghe-spokes status
+
+ ```
+ ghe-spokes status
+ ```
- Monitor replication of attachment and LFS data:
-
- ghe-storage replication-status
+
+ ```
+ ghe-storage replication-status
+ ```
- Monitor replication of Pages data:
-
- ghe-dpages replication-status
+
+ ```
+ ghe-dpages replication-status
+ ```
You can use `ghe-cluster-status` to review the overall health of your cluster. For more information, see "[Command-line utilities](/enterprise/admin/configuration/command-line-utilities#ghe-cluster-status)."
diff --git a/translations/ko-KR/content/admin/enterprise-management/increasing-storage-capacity.md b/translations/ko-KR/content/admin/enterprise-management/increasing-storage-capacity.md
index bb41f8c6021a..1b8a6164c8e9 100644
--- a/translations/ko-KR/content/admin/enterprise-management/increasing-storage-capacity.md
+++ b/translations/ko-KR/content/admin/enterprise-management/increasing-storage-capacity.md
@@ -20,6 +20,8 @@ As more users join {% data variables.product.product_location %}, you may need t
{% endnote %}
+#### Minimum requirements
+
{% data reusables.enterprise_installation.hardware-rec-table %}
### Increasing the data partition size
diff --git a/translations/ko-KR/content/admin/installation/installing-github-enterprise-server-on-google-cloud-platform.md b/translations/ko-KR/content/admin/installation/installing-github-enterprise-server-on-google-cloud-platform.md
index c45627864559..71f0fc7669a8 100644
--- a/translations/ko-KR/content/admin/installation/installing-github-enterprise-server-on-google-cloud-platform.md
+++ b/translations/ko-KR/content/admin/installation/installing-github-enterprise-server-on-google-cloud-platform.md
@@ -26,14 +26,14 @@ Before launching {% data variables.product.product_location %} on Google Cloud P
{% data variables.product.prodname_ghe_server %} is supported on the following Google Compute Engine (GCE) machine types. For more information, see [the Google Cloud Platform machine types article](https://cloud.google.com/compute/docs/machine-types).
-| | High-memory |
-| | ------------- |
-| | n1-highmem-4 |
-| | n1-highmem-8 |
-| | n1-highmem-16 |
-| | n1-highmem-32 |
-| | n1-highmem-64 |
-| | n1-highmem-96 |
+| High-memory |
+| ------------- |
+| n1-highmem-4 |
+| n1-highmem-8 |
+| n1-highmem-16 |
+| n1-highmem-32 |
+| n1-highmem-64 |
+| n1-highmem-96 |
#### Recommended machine types
@@ -54,7 +54,7 @@ Based on your user license count, we recommend these machine types.
1. Using the [gcloud compute](https://cloud.google.com/compute/docs/gcloud-compute/) command-line tool, list the public {% data variables.product.prodname_ghe_server %} images:
```shell
$ gcloud compute images list --project github-enterprise-public --no-standard-images
- ```
+ ```
2. Take note of the image name for the latest GCE image of {% data variables.product.prodname_ghe_server %}.
@@ -63,18 +63,18 @@ Based on your user license count, we recommend these machine types.
GCE virtual machines are created as a member of a network, which has a firewall. For the network associated with the {% data variables.product.prodname_ghe_server %} VM, you'll need to configure the firewall to allow the required ports listed in the table below. For more information about firewall rules on Google Cloud Platform, see the Google guide "[Firewall Rules Overview](https://cloud.google.com/vpc/docs/firewalls)."
1. Using the gcloud compute command-line tool, create the network. For more information, see "[gcloud compute networks create](https://cloud.google.com/sdk/gcloud/reference/compute/networks/create)" in the Google documentation.
- ```shell
- $ gcloud compute networks create NETWORK-NAME --subnet-mode auto
- ```
+ ```shell
+ $ gcloud compute networks create NETWORK-NAME --subnet-mode auto
+ ```
2. Create a firewall rule for each of the ports in the table below. For more information, see "[gcloud compute firewall-rules](https://cloud.google.com/sdk/gcloud/reference/compute/firewall-rules/)" in the Google documentation.
- ```shell
- $ gcloud compute firewall-rules create RULE-NAME \
- --network NETWORK-NAME \
- --allow tcp:22,tcp:25,tcp:80,tcp:122,udp:161,tcp:443,udp:1194,tcp:8080,tcp:8443,tcp:9418,icmp
- ```
- This table identifies the required ports and what each port is used for.
+ ```shell
+ $ gcloud compute firewall-rules create RULE-NAME \
+ --network NETWORK-NAME \
+ --allow tcp:22,tcp:25,tcp:80,tcp:122,udp:161,tcp:443,udp:1194,tcp:8080,tcp:8443,tcp:9418,icmp
+ ```
+ This table identifies the required ports and what each port is used for.
- {% data reusables.enterprise_installation.necessary_ports %}
+ {% data reusables.enterprise_installation.necessary_ports %}
### Allocating a static IP and assigning it to the VM
@@ -87,21 +87,21 @@ In production High Availability configurations, both primary and replica applian
To create the {% data variables.product.prodname_ghe_server %} instance, you'll need to create a GCE instance with your {% data variables.product.prodname_ghe_server %} image and attach an additional storage volume for your instance data. For more information, see "[Hardware considerations](#hardware-considerations)."
1. Using the gcloud compute command-line tool, create a data disk to use as an attached storage volume for your instance data, and configure the size based on your user license count. For more information, see "[gcloud compute disks create](https://cloud.google.com/sdk/gcloud/reference/compute/disks/create)" in the Google documentation.
- ```shell
- $ gcloud compute disks create DATA-DISK-NAME --size DATA-DISK-SIZE --type DATA-DISK-TYPE --zone ZONE
- ```
+ ```shell
+ $ gcloud compute disks create DATA-DISK-NAME --size DATA-DISK-SIZE --type DATA-DISK-TYPE --zone ZONE
+ ```
2. Then create an instance using the name of the {% data variables.product.prodname_ghe_server %} image you selected, and attach the data disk. For more information, see "[gcloud compute instances create](https://cloud.google.com/sdk/gcloud/reference/compute/instances/create)" in the Google documentation.
- ```shell
- $ gcloud compute instances create INSTANCE-NAME \
- --machine-type n1-standard-8 \
- --image GITHUB-ENTERPRISE-IMAGE-NAME \
- --disk name=DATA-DISK-NAME \
- --metadata serial-port-enable=1 \
- --zone ZONE \
- --network NETWORK-NAME \
- --image-project github-enterprise-public
- ```
+ ```shell
+ $ gcloud compute instances create INSTANCE-NAME \
+ --machine-type n1-standard-8 \
+ --image GITHUB-ENTERPRISE-IMAGE-NAME \
+ --disk name=DATA-DISK-NAME \
+ --metadata serial-port-enable=1 \
+ --zone ZONE \
+ --network NETWORK-NAME \
+ --image-project github-enterprise-public
+ ```
### Configuring the instance
diff --git a/translations/ko-KR/content/admin/overview/about-enterprise-accounts.md b/translations/ko-KR/content/admin/overview/about-enterprise-accounts.md
index 3d1804f81f01..02c92ad31de7 100644
--- a/translations/ko-KR/content/admin/overview/about-enterprise-accounts.md
+++ b/translations/ko-KR/content/admin/overview/about-enterprise-accounts.md
@@ -1,26 +1,31 @@
---
title: About enterprise accounts
-intro: 'With {% data variables.product.prodname_ghe_server %}, you can create an enterprise account to give administrators a single point of visibility and management for their billing and license usage.'
+intro: 'With {% data variables.product.product_name %}, you can use an enterprise account to give administrators a single point of visibility and management{% if enterpriseServerVersions contains currentVersion %} for billing and license usage{% endif %}.'
redirect_from:
- /enterprise/admin/installation/about-enterprise-accounts
- /enterprise/admin/overview/about-enterprise-accounts
versions:
- enterprise-server: '*'
+ enterprise-server: '>=2.20'
+ github-ae: '*'
---
-### About enterprise accounts on {% data variables.product.prodname_ghe_server %}
+### About enterprise accounts on {% data variables.product.product_name %}
-An enterprise account allows you to manage multiple {% data variables.product.prodname_dotcom %} organizations and {% data variables.product.prodname_ghe_server %} instances. Your enterprise account must have a handle, like an organization or personal account on {% data variables.product.prodname_dotcom %}. Enterprise administrators can manage settings and preferences, like:
+An enterprise account allows you to manage multiple organizations{% if enterpriseServerVersions contains currentVersion %} and {% data variables.product.prodname_ghe_server %} instances{% else %} on {% data variables.product.product_name %}{% endif %}. Your enterprise account must have a handle, like an organization or personal account on {% data variables.product.prodname_dotcom %}. Enterprise administrators can manage settings and preferences, like:
-- Member access and management (organization members, outside collaborators)
-- Billing and usage ({% data variables.product.prodname_ghe_server %} instances, user licenses, {% data variables.large_files.product_name_short %} packs)
-- Security (single sign-on, two factor authentication)
-- Requests and support bundle sharing with {% data variables.contact.enterprise_support %}
+- Member access and management (organization members, outside collaborators){% if enterpriseServerVersions contains currentVersion %}
+- Billing and usage ({% data variables.product.prodname_ghe_server %} instances, user licenses, {% data variables.large_files.product_name_short %} packs){% endif %}
+- Security{% if enterpriseServerVersions contains currentVersion %}(single sign-on, two factor authentication)
+- Requests {% if enterpriseServerVersions contains currentVersion %}and support bundle sharing {% endif %}with {% data variables.contact.enterprise_support %}{% endif %}
-{% data reusables.enterprise-accounts.enterprise-accounts-billing %}
+{% if enterpriseServerVersions contains currentVersion %}{% data reusables.enterprise-accounts.enterprise-accounts-billing %} For more information about managing your {% data variables.product.prodname_ghe_cloud %} subscription, see "[Viewing the subscription and usage for your enterprise account](/articles/viewing-the-subscription-and-usage-for-your-enterprise-account)." {% endif %}For more information about managing your {% data variables.product.product_name %} billing settings, see "[Managing billing for your enterprise](/admin/overview/managing-billing-for-your-enterprise)."
+
+{% if enterpriseServerVersions contains currentVersion %}
For more information about the differences between {% data variables.product.prodname_ghe_cloud %} and {% data variables.product.prodname_ghe_server %}, see "[{% data variables.product.prodname_dotcom %}'s products](/articles/githubs-products)." To upgrade to {% data variables.product.prodname_enterprise %} or to get started with an enterprise account, contact {% data variables.contact.contact_enterprise_sales %}.
### Managing {% data variables.product.prodname_ghe_server %} licenses linked to your enterprise account
{% data reusables.enterprise-accounts.admin-managing-licenses %}
+
+{% endif %}
diff --git a/translations/ko-KR/content/admin/packages/configuring-third-party-storage-for-packages.md b/translations/ko-KR/content/admin/packages/configuring-third-party-storage-for-packages.md
index 2a7e32e8c90d..82e18d48a737 100644
--- a/translations/ko-KR/content/admin/packages/configuring-third-party-storage-for-packages.md
+++ b/translations/ko-KR/content/admin/packages/configuring-third-party-storage-for-packages.md
@@ -13,7 +13,7 @@ versions:
{% data variables.product.prodname_registry %} on {% data variables.product.prodname_ghe_server %} uses external blob storage to store your packages. The amount of storage required depends on your usage of {% data variables.product.prodname_registry %}.
-At this time, {% data variables.product.prodname_registry %} supports blob storage with Amazon Web Services (AWS) S3. MinIO is also supported, but configuration is not currently implemented in the {% data variables.product.product_name %} interface. You can use MinIO for storage by following the instructions for AWS S3, entering the analogous information for your MinIO configuration.
+At this time, {% data variables.product.prodname_registry %} supports blob storage with Amazon Web Services (AWS) S3. MinIO is also supported, but configuration is not currently implemented in the {% data variables.product.product_name %} interface. You can use MinIO for storage by following the instructions for AWS S3, entering the analogous information for your MinIO configuration. Before configuring third-party storage for {% data variables.product.prodname_registry %} on {% data variables.product.prodname_dotcom %}, you must set up a bucket with your third-party storage provider. For more information on installing and running a MinIO bucket to use with {% data variables.product.prodname_registry %}, see the "[Quickstart for configuring MinIO storage](/admin/packages/quickstart-for-configuring-minio-storage)."
For the best experience, we recommend using a dedicated bucket for {% data variables.product.prodname_registry %}, separate from the bucket you use for {% data variables.product.prodname_actions %} storage.
diff --git a/translations/ko-KR/content/admin/packages/index.md b/translations/ko-KR/content/admin/packages/index.md
index 2c1a7b4d0c1f..f73f90725995 100644
--- a/translations/ko-KR/content/admin/packages/index.md
+++ b/translations/ko-KR/content/admin/packages/index.md
@@ -10,5 +10,6 @@ versions:
{% data reusables.package_registry.packages-ghes-release-stage %}
{% link_with_intro /enabling-github-packages-for-your-enterprise %}
+{% link_with_intro /quickstart-for-configuring-minio-storage %}
{% link_with_intro /configuring-packages-support-for-your-enterprise %}
{% link_with_intro /configuring-third-party-storage-for-packages %}
diff --git a/translations/ko-KR/content/admin/packages/quickstart-for-configuring-minio-storage.md b/translations/ko-KR/content/admin/packages/quickstart-for-configuring-minio-storage.md
new file mode 100644
index 000000000000..40d028ef6a98
--- /dev/null
+++ b/translations/ko-KR/content/admin/packages/quickstart-for-configuring-minio-storage.md
@@ -0,0 +1,133 @@
+---
+title: Quickstart for configuring MinIO storage
+intro: 'Set up MinIO as a storage provider for using {% data variables.product.prodname_registry %} on your enterprise.'
+versions:
+ enterprise-server: '>=2.22'
+---
+
+{% data reusables.package_registry.packages-ghes-release-stage %}
+
+Before you can enable and configure {% data variables.product.prodname_registry %} on {% data variables.product.product_location_enterprise %}, you need to prepare your third-party storage solution.
+
+MinIO offers object storage with support for the S3 API and {% data variables.product.prodname_registry %} on your enterprise.
+
+This quickstart shows you how to set up MinIO using Docker for use with {% data variables.product.prodname_registry %} but you have other options for managing MinIO besides Docker. For more information about MinIO, see the official [MinIO docs](https://docs.min.io/).
+
+### 1. Choose a MinIO mode for your needs
+
+| MinIO mode | Optimized for | Storage infrastructure required |
+| ----------------------------------------------- | ------------------------------ | ------------------------------------ |
+| Standalone MinIO (on a single host) | Fast setup | N/A |
+| MinIO as a NAS gateway | NAS (Network-attached storage) | NAS devices |
+| Clustered MinIO (also called Distributed MinIO) | Data security | Storage servers running in a cluster |
+
+For more information about your options, see the official [MinIO docs](https://docs.min.io/).
+
+### 2. Install, run, and sign in to MinIO
+
+1. Set up your preferred environment variables for MinIO.
+
+ These examples use `MINIO_DIR`:
+ ```shell
+ $ export MINIO_DIR=$(pwd)/minio
+ $ mkdir -p $MINIO_DIR
+ ```
+
+2. Install MinIO.
+
+ ```shell
+ $ docker pull minio/minio
+ ```
+ For more information, see the official "[MinIO Quickstart Guide](https://docs.min.io/docs/minio-quickstart-guide)."
+
+3. Sign in to MinIO using your MinIO access key and secret.
+
+ {% linux %}
+ ```shell
+ $ export MINIO_ACCESS_KEY=$(cat /dev/urandom | tr -dc 'a-zA-Z0-9' | fold -w 32 | head -n 1)
+ # this one is actually a secret, so careful
+ $ export MINIO_SECRET_KEY=$(cat /dev/urandom | tr -dc 'a-zA-Z0-9' | fold -w 32 | head -n 1)
+ ```
+ {% endlinux %}
+
+ {% mac %}
+ ```shell
+ $ export MINIO_ACCESS_KEY=$(cat /dev/urandom | LC_CTYPE=C tr -dc 'a-zA-Z0-9' | fold -w 32 | head -n 1)
+ # this one is actually a secret, so careful
+ $ export MINIO_SECRET_KEY=$(cat /dev/urandom | LC_CTYPE=C tr -dc 'a-zA-Z0-9' | fold -w 32 | head -n 1)
+ ```
+ {% endmac %}
+
+ You can access your MinIO keys using the environment variables:
+
+ ```shell
+ $ echo $MINIO_ACCESS_KEY
+ $ echo $MINIO_SECRET_KEY
+ ```
+
+4. Run MinIO in your chosen mode.
+
+ * Run MinIO using Docker on a single host:
+
+ ```shell
+ $ docker run -p 9000:9000 \
+ -v $MINIO_DIR:/data \
+ -e "MINIO_ACCESS_KEY=$MINIO_ACCESS_KEY" \
+ -e "MINIO_SECRET_KEY=$MINIO_SECRET_KEY" \
+ minio/minio server /data
+ ```
+
+ For more information, see "[MinIO Docker Quickstart guide](https://docs.min.io/docs/minio-docker-quickstart-guide.html)."
+
+ * Run MinIO using Docker as a NAS gateway:
+
+ This setup is useful for deployments where there is already a NAS you want to use as the backup storage for {% data variables.product.prodname_registry %}.
+
+ ```shell
+ $ docker run -p 9000:9000 \
+ -v $MINIO_DIR:/data \
+ -e "MINIO_ACCESS_KEY=$MINIO_ACCESS_KEY" \
+ -e "MINIO_SECRET_KEY=$MINIO_SECRET_KEY" \
+ minio/minio gateway nas /data
+ ```
+
+ For more information, see "[MinIO Gateway for NAS](https://docs.min.io/docs/minio-gateway-for-nas.html)."
+
+ * Run MinIO using Docker as a cluster. This MinIO deployment uses several hosts and MinIO's erasure coding for the strongest data protection. To run MinIO in a cluster mode, see the "[Distributed MinIO Quickstart Guide](https://docs.min.io/docs/distributed-minio-quickstart-guide.html).
+
+### 3. Create your MinIO bucket for {% data variables.product.prodname_registry %}
+
+1. Install the MinIO client.
+
+ ```shell
+ $ docker pull minio/mc
+ ```
+
+2. Create a bucket with a host URL that {% data variables.product.prodname_ghe_server %} can access.
+
+ * Local deployments example:
+
+ ```shell
+ $ export MC_HOST_minio="http://${MINIO_ACCESS_KEY}:${MINIO_SECRET_KEY} @localhost:9000"
+ $ docker run minio/mc BUCKET-NAME
+ ```
+
+ This example can be used for MinIO standalone or MinIO as a NAS gateway.
+
+ * Clustered deployments example:
+
+ ```shell
+ $ export MC_HOST_minio="http://${MINIO_ACCESS_KEY}:${MINIO_SECRET_KEY} @minioclustername.example.com:9000"
+ $ docker run minio/mc mb packages
+ ```
+
+
+### 다음 단계
+
+To finish configuring storage for {% data variables.product.prodname_registry %}, you'll need to copy the MinIO storage URL:
+
+ ```
+ echo "http://${MINIO_ACCESS_KEY}:${MINIO_SECRET_KEY}@minioclustername.example.com:9000"
+ ```
+
+For the next steps, see "[Configuring third-party storage for packages](/admin/packages/configuring-third-party-storage-for-packages)."
\ No newline at end of file
diff --git a/translations/ko-KR/content/admin/policies/creating-a-pre-receive-hook-environment.md b/translations/ko-KR/content/admin/policies/creating-a-pre-receive-hook-environment.md
index b082fd2e9586..0f2b2aabfd50 100644
--- a/translations/ko-KR/content/admin/policies/creating-a-pre-receive-hook-environment.md
+++ b/translations/ko-KR/content/admin/policies/creating-a-pre-receive-hook-environment.md
@@ -21,10 +21,10 @@ You can use a Linux container management tool to build a pre-receive hook enviro
{% data reusables.linux.ensure-docker %}
2. Create the file `Dockerfile.alpine-3.3` that contains this information:
- ```
- FROM gliderlabs/alpine:3.3
- RUN apk add --no-cache git bash
- ```
+ ```
+ FROM gliderlabs/alpine:3.3
+ RUN apk add --no-cache git bash
+ ```
3. From the working directory that contains `Dockerfile.alpine-3.3`, build an image:
```shell
@@ -36,37 +36,37 @@ You can use a Linux container management tool to build a pre-receive hook enviro
> ---> Using cache
> ---> 0250ab3be9c5
> Successfully built 0250ab3be9c5
- ```
+ ```
4. Create a container:
```shell
$ docker create --name pre-receive.alpine-3.3 pre-receive.alpine-3.3 /bin/true
- ```
+ ```
5. Export the Docker container to a `gzip` compressed `tar` file:
```shell
$ docker export pre-receive.alpine-3.3 | gzip > alpine-3.3.tar.gz
- ```
+ ```
- This file `alpine-3.3.tar.gz` is ready to be uploaded to the {% data variables.product.prodname_ghe_server %} appliance.
+ This file `alpine-3.3.tar.gz` is ready to be uploaded to the {% data variables.product.prodname_ghe_server %} appliance.
### Creating a pre-receive hook environment using chroot
1. Create a Linux `chroot` environment.
2. Create a `gzip` compressed `tar` file of the `chroot` directory.
- ```shell
- $ cd /path/to/chroot
- $ tar -czf /path/to/pre-receive-environment.tar.gz .
+ ```shell
+ $ cd /path/to/chroot
+ $ tar -czf /path/to/pre-receive-environment.tar.gz .
```
- {% note %}
+ {% note %}
- **참고:**
- - Do not include leading directory paths of files within the tar archive, such as `/path/to/chroot`.
- - `/bin/sh` must exist and be executable, as the entry point into the chroot environment.
- - Unlike traditional chroots, the `dev` directory is not required by the chroot environment for pre-receive hooks.
+ **참고:**
+ - Do not include leading directory paths of files within the tar archive, such as `/path/to/chroot`.
+ - `/bin/sh` must exist and be executable, as the entry point into the chroot environment.
+ - Unlike traditional chroots, the `dev` directory is not required by the chroot environment for pre-receive hooks.
- {% endnote %}
+ {% endnote %}
For more information about creating a chroot environment see "[Chroot](https://wiki.debian.org/chroot)" from the *Debian Wiki*, "[BasicChroot](https://help.ubuntu.com/community/BasicChroot)" from the *Ubuntu Community Help Wiki*, or "[Installing Alpine Linux in a chroot](http://wiki.alpinelinux.org/wiki/Installing_Alpine_Linux_in_a_chroot)" from the *Alpine Linux Wiki*.
@@ -89,4 +89,4 @@ For more information about creating a chroot environment see "[Chroot](https://w
```shell
admin@ghe-host:~$ ghe-hook-env-create AlpineTestEnv /home/admin/alpine-3.3.tar.gz
> Pre-receive hook environment 'AlpineTestEnv' (2) has been created.
- ```
+ ```
diff --git a/translations/ko-KR/content/admin/policies/creating-a-pre-receive-hook-script.md b/translations/ko-KR/content/admin/policies/creating-a-pre-receive-hook-script.md
index a34f36223faf..947b9c719155 100644
--- a/translations/ko-KR/content/admin/policies/creating-a-pre-receive-hook-script.md
+++ b/translations/ko-KR/content/admin/policies/creating-a-pre-receive-hook-script.md
@@ -70,19 +70,19 @@ We recommend consolidating hooks to a single repository. If the consolidated hoo
```shell
$ sudo chmod +x SCRIPT_FILE.sh
- ```
- For Windows users, ensure the scripts have execute permissions:
+ ```
+ For Windows users, ensure the scripts have execute permissions:
- ```shell
- git update-index --chmod=+x SCRIPT_FILE.sh
- ```
+ ```shell
+ git update-index --chmod=+x SCRIPT_FILE.sh
+ ```
2. Commit and push to your designated pre-receive hooks repository on the {% data variables.product.prodname_ghe_server %} instance.
```shell
$ git commit -m "YOUR COMMIT MESSAGE"
$ git push
- ```
+ ```
3. [Create the pre-receive hook](/enterprise/{{ currentVersion }}/admin/guides/developer-workflow/managing-pre-receive-hooks-on-the-github-enterprise-server-appliance/#creating-pre-receive-hooks) on the {% data variables.product.prodname_ghe_server %} instance.
@@ -93,40 +93,40 @@ You can test a pre-receive hook script locally before you create or update it on
2. Create a file called `Dockerfile.dev` containing:
- ```
- FROM gliderlabs/alpine:3.3
- RUN \
- apk add --no-cache git openssh bash && \
- ssh-keygen -A && \
- sed -i "s/#AuthorizedKeysFile/AuthorizedKeysFile/g" /etc/ssh/sshd_config && \
- adduser git -D -G root -h /home/git -s /bin/bash && \
- passwd -d git && \
- su git -c "mkdir /home/git/.ssh && \
- ssh-keygen -t ed25519 -f /home/git/.ssh/id_ed25519 -P '' && \
- mv /home/git/.ssh/id_ed25519.pub /home/git/.ssh/authorized_keys && \
- mkdir /home/git/test.git && \
- git --bare init /home/git/test.git"
-
- VOLUME ["/home/git/.ssh", "/home/git/test.git/hooks"]
- WORKDIR /home/git
-
- CMD ["/usr/sbin/sshd", "-D"]
- ```
+ ```
+ FROM gliderlabs/alpine:3.3
+ RUN \
+ apk add --no-cache git openssh bash && \
+ ssh-keygen -A && \
+ sed -i "s/#AuthorizedKeysFile/AuthorizedKeysFile/g" /etc/ssh/sshd_config && \
+ adduser git -D -G root -h /home/git -s /bin/bash && \
+ passwd -d git && \
+ su git -c "mkdir /home/git/.ssh && \
+ ssh-keygen -t ed25519 -f /home/git/.ssh/id_ed25519 -P '' && \
+ mv /home/git/.ssh/id_ed25519.pub /home/git/.ssh/authorized_keys && \
+ mkdir /home/git/test.git && \
+ git --bare init /home/git/test.git"
+
+ VOLUME ["/home/git/.ssh", "/home/git/test.git/hooks"]
+ WORKDIR /home/git
+
+ CMD ["/usr/sbin/sshd", "-D"]
+ ```
3. Create a test pre-receive script called `always_reject.sh`. This example script will reject all pushes, which is useful for locking a repository:
- ```
- #!/usr/bin/env bash
+ ```
+ #!/usr/bin/env bash
- echo "error: rejecting all pushes"
- exit 1
- ```
+ echo "error: rejecting all pushes"
+ exit 1
+ ```
4. Ensure the `always_reject.sh` scripts has execute permissions:
```shell
$ chmod +x always_reject.sh
- ```
+ ```
5. From the directory containing `Dockerfile.dev`, build an image:
@@ -149,32 +149,32 @@ You can test a pre-receive hook script locally before you create or update it on
....truncated output....
> Initialized empty Git repository in /home/git/test.git/
> Successfully built dd8610c24f82
- ```
+ ```
6. Run a data container that contains a generated SSH key:
```shell
$ docker run --name data pre-receive.dev /bin/true
- ```
+ ```
7. Copy the test pre-receive hook `always_reject.sh` into the data container:
```shell
$ docker cp always_reject.sh data:/home/git/test.git/hooks/pre-receive
- ```
+ ```
8. Run an application container that runs `sshd` and executes the hook. Take note of the container id that is returned:
```shell
$ docker run -d -p 52311:22 --volumes-from data pre-receive.dev
> 7f888bc700b8d23405dbcaf039e6c71d486793cad7d8ae4dd184f4a47000bc58
- ```
+ ```
9. Copy the generated SSH key from the data container to the local machine:
```shell
$ docker cp data:/home/git/.ssh/id_ed25519 .
- ```
+ ```
10. Modify the remote of a test repository and push to the `test.git` repo within the Docker container. This example uses `git@github.com:octocat/Hello-World.git` but you can use any repo you want. This example assumes your local machine (127.0.0.1) is binding port 52311, but you can use a different IP address if docker is running on a remote machine.
@@ -193,9 +193,9 @@ You can test a pre-receive hook script locally before you create or update it on
> To git@192.168.99.100:test.git
> ! [remote rejected] main -> main (pre-receive hook declined)
> error: failed to push some refs to 'git@192.168.99.100:test.git'
- ```
+ ```
- Notice that the push was rejected after executing the pre-receive hook and echoing the output from the script.
+ Notice that the push was rejected after executing the pre-receive hook and echoing the output from the script.
### 더 읽을거리
- "[Customizing Git - An Example Git-Enforced Policy](https://git-scm.com/book/en/v2/Customizing-Git-An-Example-Git-Enforced-Policy)" from the *Pro Git website*
diff --git a/translations/ko-KR/content/admin/policies/enforcing-repository-management-policies-in-your-enterprise.md b/translations/ko-KR/content/admin/policies/enforcing-repository-management-policies-in-your-enterprise.md
index b1ad1c38b988..9fed38be0771 100644
--- a/translations/ko-KR/content/admin/policies/enforcing-repository-management-policies-in-your-enterprise.md
+++ b/translations/ko-KR/content/admin/policies/enforcing-repository-management-policies-in-your-enterprise.md
@@ -34,7 +34,7 @@ versions:
Each time someone creates a new repository on your enterprise, that person must choose a visibility for the repository. When you configure a default visibility setting for the enterprise, you choose which visibility is selected by default. For more information on repository visibility, see "[About repository visibility](/github/creating-cloning-and-archiving-repositories/about-repository-visibility)."
-If a site administrator disallows members from creating certain types of repositories, members will not be able to create that type of repository even if the visibility setting defaults to that type. For more information, see "[Setting a policy for repository creation](#setting-a-policy-for-repository-creation)."
+If an enterprise owner disallows members from creating certain types of repositories, members will not be able to create that type of repository even if the visibility setting defaults to that type. For more information, see "[Setting a policy for repository creation](#setting-a-policy-for-repository-creation)."
{% data reusables.enterprise-accounts.access-enterprise %}
{% if currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
@@ -49,9 +49,9 @@ If a site administrator disallows members from creating certain types of reposit
### Setting a policy for changing a repository's visibility
-When you prevent members from changing repository visibility, only site administrators have the ability to make public repositories private or make private repositories public.
+When you prevent members from changing repository visibility, only enterprise owners can change the visibility of a repository.
-If a site administrator has restricted repository creation to organization owners only, then members will not be able to change repository visibility. If a site administrator has restricted member repository creation to private repositories only, then members will only be able to change repositories from public to private. For more information, see "[Setting a policy for repository creation](#setting-a-policy-for-repository-creation)."
+If an enterprise owner has restricted repository creation to organization owners only, then members will not be able to change repository visibility. If an enterprise owner has restricted member repository creation to private repositories only, then members will only be able to change the visibility of a repository to private. For more information, see "[Setting a policy for repository creation](#setting-a-policy-for-repository-creation)."
{% data reusables.enterprise-accounts.access-enterprise %}
{% data reusables.enterprise-accounts.policies-tab %}
@@ -75,6 +75,15 @@ If a site administrator has restricted repository creation to organization owner
6. Under "Repository creation", use the drop-down menu and choose a policy. 
{% endif %}
+### Enforcing a policy on forking private or internal repositories
+
+Across all organizations owned by your enterprise, you can allow people with access to a private or internal repository to fork the repository, never allow forking of private or internal repositories, or allow owners to administer the setting on the organization level.
+
+{% data reusables.enterprise-accounts.access-enterprise %}
+{% data reusables.enterprise-accounts.policies-tab %}
+3. On the **Repository policies** tab, under "Repository forking", review the information about changing the setting. {% data reusables.enterprise-accounts.view-current-policy-config-orgs %}
+4. Under "Repository forking", use the drop-down menu and choose a policy. 
+
### Setting a policy for repository deletion and transfer
{% data reusables.enterprise-accounts.access-enterprise %}
@@ -166,6 +175,8 @@ You can override the default inherited settings by configuring the settings for
- **Block to the default branch** to only block force pushes to the default branch. 
6. Optionally, select **Enforce on all repositories** to override repository-specific settings. Note that this will **not** override an enterprise-wide policy. 
+{% if enterpriseServerVersions contains currentVersion %}
+
### Configuring anonymous Git read access
{% data reusables.enterprise_user_management.disclaimer-for-git-read-access %}
@@ -192,7 +203,6 @@ If necessary, you can prevent repository administrators from changing anonymous
4. Under "Anonymous Git read access", use the drop-down menu, and click **Enabled**. 
3. Optionally, to prevent repository admins from changing anonymous Git read access settings in all repositories on your enterprise, select **Prevent repository admins from changing anonymous Git read access**. 
-{% if enterpriseServerVersions contains currentVersion %}
#### Setting anonymous Git read access for a specific repository
{% data reusables.enterprise_site_admin_settings.access-settings %}
@@ -203,6 +213,7 @@ If necessary, you can prevent repository administrators from changing anonymous
6. Under "Danger Zone", next to "Enable Anonymous Git read access", click **Enable**. 
7. Review the changes. To confirm, click **Yes, enable anonymous Git read access.** 
8. Optionally, to prevent repository admins from changing this setting for this repository, select **Prevent repository admins from changing anonymous Git read access**. 
+
{% endif %}
{% if currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
diff --git a/translations/ko-KR/content/admin/release-notes.md b/translations/ko-KR/content/admin/release-notes.md
new file mode 100644
index 000000000000..d312be458ea8
--- /dev/null
+++ b/translations/ko-KR/content/admin/release-notes.md
@@ -0,0 +1,8 @@
+---
+title: Release notes
+intro: The release notes for {{ allVersions[currentVersion].versionTitle }}.
+layout: release-notes
+versions:
+ enterprise-server: '*'
+---
+
diff --git a/translations/ko-KR/content/admin/user-management/audited-actions.md b/translations/ko-KR/content/admin/user-management/audited-actions.md
index 6d5e10a80284..74a877f3b0c3 100644
--- a/translations/ko-KR/content/admin/user-management/audited-actions.md
+++ b/translations/ko-KR/content/admin/user-management/audited-actions.md
@@ -36,12 +36,12 @@ versions:
#### Enterprise configuration settings
-| 이름 | 설명 |
-| -------------------------------------------------------:| ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `business.update_member_repository_creation_permission` | A site admin restricts repository creation in organizations in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#setting-a-policy-for-repository-creation)." |
-| `business.clear_members_can_create_repos` | A site admin clears a restriction on repository creation in organizations in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#setting-a-policy-for-repository-creation)." |
-| `enterprise.config.lock_anonymous_git_access` | A site admin locks anonymous Git read access to prevent repository admins from changing existing anonymous Git read access settings for repositories in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#configuring-anonymous-git-read-access)." |
-| `enterprise.config.unlock_anonymous_git_access` | A site admin unlocks anonymous Git read access to allow repository admins to change existing anonymous Git read access settings for repositories in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#configuring-anonymous-git-read-access)." |
+| 이름 | 설명 |
+| -------------------------------------------------------:| -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `business.update_member_repository_creation_permission` | A site admin restricts repository creation in organizations in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#setting-a-policy-for-repository-creation)." |
+| `business.clear_members_can_create_repos` | A site admin clears a restriction on repository creation in organizations in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#setting-a-policy-for-repository-creation)."{% if enterpriseServerVersions contains currentVersion %}
+| `enterprise.config.lock_anonymous_git_access` | A site admin locks anonymous Git read access to prevent repository admins from changing existing anonymous Git read access settings for repositories in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#configuring-anonymous-git-read-access)." |
+| `enterprise.config.unlock_anonymous_git_access` | A site admin unlocks anonymous Git read access to allow repository admins to change existing anonymous Git read access settings for repositories in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#configuring-anonymous-git-read-access)."{% endif %}
#### Issues and pull requests
@@ -77,23 +77,23 @@ versions:
#### Repositories
-| 이름 | 설명 |
-| ------------------------------------------:| -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `repo.access` | A private repository was made public, or a public repository was made private. |
-| `repo.archive` | A repository was archived. For more information, see "[Archiving a {% data variables.product.prodname_dotcom %} repository](/github/creating-cloning-and-archiving-repositories/archiving-a-github-repository)." |
-| `repo.add_member` | A collaborator was added to a repository. |
-| `repo.config` | A site admin blocked force pushes. For more information, see [Blocking force pushes to a repository](/enterprise/{{ currentVersion }}/admin/guides/developer-workflow/blocking-force-pushes-to-a-repository/) to a repository. |
-| `repo.create` | A repository was created. |
-| `repo.destroy` | A repository was deleted. |
-| `repo.remove_member` | A collaborator was removed from a repository. |
-| `repo.rename` | A repository was renamed. |
-| `repo.transfer` | A user accepted a request to receive a transferred repository. |
-| `repo.transfer_start` | A user sent a request to transfer a repository to another user or organization. |
-| `repo.unarchive` | A repository was unarchived. For more information, see "[Archiving a {% data variables.product.prodname_dotcom %} repository](/github/creating-cloning-and-archiving-repositories/archiving-a-github-repository)." |
-| `repo.config.disable_anonymous_git_access` | Anonymous Git read access is disabled for a public repository. For more information, see "[Enabling anonymous Git read access for a repository](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)." |
-| `repo.config.enable_anonymous_git_access` | Anonymous Git read access is enabled for a public repository. For more information, see "[Enabling anonymous Git read access for a repository](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)." |
-| `repo.config.lock_anonymous_git_access` | A repository's anonymous Git read access setting is locked, preventing repository administrators from changing (enabling or disabling) this setting. For more information, see "[Preventing users from changing anonymous Git read access](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)." |
-| `repo.config.unlock_anonymous_git_access` | A repository's anonymous Git read access setting is unlocked, allowing repository administrators to change (enable or disable) this setting. For more information, see "[Preventing users from changing anonymous Git read access](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)." |
+| 이름 | 설명 |
+| ------------------------------------------:| ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `repo.access` | The visibility of a repository changed to private{% if enterpriseServerVersions contains currentVersion %}, public,{% endif %} or internal. |
+| `repo.archive` | A repository was archived. For more information, see "[Archiving a {% data variables.product.prodname_dotcom %} repository](/github/creating-cloning-and-archiving-repositories/archiving-a-github-repository)." |
+| `repo.add_member` | A collaborator was added to a repository. |
+| `repo.config` | A site admin blocked force pushes. For more information, see [Blocking force pushes to a repository](/enterprise/{{ currentVersion }}/admin/guides/developer-workflow/blocking-force-pushes-to-a-repository/) to a repository. |
+| `repo.create` | A repository was created. |
+| `repo.destroy` | A repository was deleted. |
+| `repo.remove_member` | A collaborator was removed from a repository. |
+| `repo.rename` | A repository was renamed. |
+| `repo.transfer` | A user accepted a request to receive a transferred repository. |
+| `repo.transfer_start` | A user sent a request to transfer a repository to another user or organization. |
+| `repo.unarchive` | A repository was unarchived. For more information, see "[Archiving a {% data variables.product.prodname_dotcom %} repository](/github/creating-cloning-and-archiving-repositories/archiving-a-github-repository)."{% if enterpriseServerVersions contains currentVersion %}
+| `repo.config.disable_anonymous_git_access` | Anonymous Git read access is disabled for a repository. For more information, see "[Enabling anonymous Git read access for a repository](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)." |
+| `repo.config.enable_anonymous_git_access` | Anonymous Git read access is enabled for a repository. For more information, see "[Enabling anonymous Git read access for a repository](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)." |
+| `repo.config.lock_anonymous_git_access` | A repository's anonymous Git read access setting is locked, preventing repository administrators from changing (enabling or disabling) this setting. For more information, see "[Preventing users from changing anonymous Git read access](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)." |
+| `repo.config.unlock_anonymous_git_access` | A repository's anonymous Git read access setting is unlocked, allowing repository administrators to change (enable or disable) this setting. For more information, see "[Preventing users from changing anonymous Git read access](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)."{% endif %}
#### Site admin tools
diff --git a/translations/ko-KR/content/admin/user-management/managing-organizations-in-your-enterprise.md b/translations/ko-KR/content/admin/user-management/managing-organizations-in-your-enterprise.md
index 46c6cec1ac63..0bccc9095bd8 100644
--- a/translations/ko-KR/content/admin/user-management/managing-organizations-in-your-enterprise.md
+++ b/translations/ko-KR/content/admin/user-management/managing-organizations-in-your-enterprise.md
@@ -5,7 +5,7 @@ redirect_from:
- /enterprise/admin/categories/admin-bootcamp/
- /enterprise/admin/user-management/organizations-and-teams
- /enterprise/admin/user-management/managing-organizations-in-your-enterprise
-intro: 'Organizations are great for creating distinct groups of users within your company, such as divisions or groups working on similar projects. Public repositories that belong to an organization are accessible to users in other organizations, while private repositories are inaccessible to anyone but members of the organization.'
+intro: 'Organizations are great for creating distinct groups of users within your company, such as divisions or groups working on similar projects. {% if currentVersion == "github-ae@latest" %}Internal{% else %}Public and internal{% endif %} repositories that belong to an organization are accessible to users in other organizations, while private repositories are inaccessible to anyone but members of the organization that are granted access.'
mapTopic: true
versions:
enterprise-server: '*'
diff --git a/translations/ko-KR/content/developers/apps/authorizing-oauth-apps.md b/translations/ko-KR/content/developers/apps/authorizing-oauth-apps.md
index 17ae20fc1f15..04007cdc3e30 100644
--- a/translations/ko-KR/content/developers/apps/authorizing-oauth-apps.md
+++ b/translations/ko-KR/content/developers/apps/authorizing-oauth-apps.md
@@ -114,11 +114,13 @@ curl -H "Authorization: token OAUTH-TOKEN" {% data variables.product.api_url_pre
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
### Device flow
+{% if currentVersion ver_lt "enterprise-server@3.1" %}
{% note %}
-**Note:** The device flow is in public beta and subject to change.{% if currentVersion == "free-pro-team@latest" %} To enable this beta feature, see "[Activating beta features for apps](/developers/apps/activating-beta-features-for-apps)."{% endif %}
+**Note:** The device flow is in public beta and subject to change.
{% endnote %}
+{% endif %}
The device flow allows you to authorize users for a headless app, such as a CLI tool or Git credential manager.
diff --git a/translations/ko-KR/content/developers/apps/identifying-and-authorizing-users-for-github-apps.md b/translations/ko-KR/content/developers/apps/identifying-and-authorizing-users-for-github-apps.md
index a1d18a0d1453..2ca154a0014e 100644
--- a/translations/ko-KR/content/developers/apps/identifying-and-authorizing-users-for-github-apps.md
+++ b/translations/ko-KR/content/developers/apps/identifying-and-authorizing-users-for-github-apps.md
@@ -123,11 +123,13 @@ curl -H "Authorization: token OAUTH-TOKEN" {% data variables.product.api_url_pre
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
### Device flow
+{% if currentVersion ver_lt "enterprise-server@3.1" %}
{% note %}
-**Note:** The device flow is in public beta and subject to change.{% if currentVersion == "free-pro-team@latest" %} To enable this beta feature, see "[Activating beta features for apps](/developers/apps/activating-beta-features-for-apps)."{% endif %}
+**Note:** The device flow is in public beta and subject to change.
{% endnote %}
+{% endif %}
The device flow allows you to authorize users for a headless app, such as a CLI tool or Git credential manager.
diff --git a/translations/ko-KR/content/developers/webhooks-and-events/webhook-events-and-payloads.md b/translations/ko-KR/content/developers/webhooks-and-events/webhook-events-and-payloads.md
index 0b36a7b6c5db..9606a2ea2751 100644
--- a/translations/ko-KR/content/developers/webhooks-and-events/webhook-events-and-payloads.md
+++ b/translations/ko-KR/content/developers/webhooks-and-events/webhook-events-and-payloads.md
@@ -1288,7 +1288,7 @@ The event’s actor is the [user](/v3/users/) who starred a repository, and the
{{ webhookPayloadsForCurrentVersion.watch.started }}
-{% if currentVersion == "free-pro-team@latest" %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
### workflow_dispatch
This event occurs when someone triggers a workflow run on GitHub or sends a `POST` request to the "[Create a workflow dispatch event](/rest/reference/actions/#create-a-workflow-dispatch-event)" endpoint. For more information, see "[Events that trigger workflows](/actions/reference/events-that-trigger-workflows#workflow_dispatch)."
@@ -1302,6 +1302,7 @@ This event occurs when someone triggers a workflow run on GitHub or sends a `POS
{{ webhookPayloadsForCurrentVersion.workflow_dispatch }}
{% endif %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %}
### workflow_run
When a {% data variables.product.prodname_actions %} workflow run is requested or completed. For more information, see "[Events that trigger workflows](/actions/reference/events-that-trigger-workflows#workflow_run)."
@@ -1322,3 +1323,4 @@ When a {% data variables.product.prodname_actions %} workflow run is requested o
#### Webhook payload example
{{ webhookPayloadsForCurrentVersion.workflow_run }}
+{% endif %}
diff --git a/translations/ko-KR/content/github/administering-a-repository/about-secret-scanning.md b/translations/ko-KR/content/github/administering-a-repository/about-secret-scanning.md
index 12e54afd4af9..32380d4d9738 100644
--- a/translations/ko-KR/content/github/administering-a-repository/about-secret-scanning.md
+++ b/translations/ko-KR/content/github/administering-a-repository/about-secret-scanning.md
@@ -18,6 +18,8 @@ Service providers can partner with {% data variables.product.company_short %} to
### About {% data variables.product.prodname_secret_scanning %} for public repositories
+ {% data variables.product.prodname_secret_scanning_caps %} is automatically enabled on public repositories, where it scans code for secrets, to check for known secret formats. When a match of your secret format is found in a public repository, {% data variables.product.company_short %} doesn't publicly disclose the information as an alert, but instead sends a payload to an HTTP endpoint of your choice. For an overview of how secret scanning works on public repositories, see "[Secret scanning](/developers/overview/secret-scanning)."
+
When you push to a public repository, {% data variables.product.product_name %} scans the content of the commits for secrets. If you switch a private repository to public, {% data variables.product.product_name %} scans the entire repository for secrets.
When {% data variables.product.prodname_secret_scanning %} detects a set of credentials, we notify the service provider who issued the secret. The service provider validates the credential and then decides whether they should revoke the secret, issue a new secret, or reach out to you directly, which will depend on the associated risks to you or the service provider.
@@ -65,6 +67,8 @@ When {% data variables.product.prodname_secret_scanning %} detects a set of cred
{% data reusables.secret-scanning.beta %}
+If you're a repository administrator or an organization owner, you can enable {% data variables.product.prodname_secret_scanning %} for private repositories that are owned by organizations. You can enable {% data variables.product.prodname_secret_scanning %} for all your repositories, or for all new repositories within your organization. {% data variables.product.prodname_secret_scanning_caps %} is not available for user account-owned private repositories. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository)" and "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)."
+
When you push commits to a private repository with {% data variables.product.prodname_secret_scanning %} enabled, {% data variables.product.product_name %} scans the contents of the commits for secrets.
When {% data variables.product.prodname_secret_scanning %} detects a secret in a private repository, {% data variables.product.prodname_dotcom %} sends alerts.
@@ -73,6 +77,8 @@ When {% data variables.product.prodname_secret_scanning %} detects a secret in a
- {% data variables.product.prodname_dotcom %} displays an alert in the repository. For more information, see "[Managing alerts from {% data variables.product.prodname_secret_scanning %}](/github/administering-a-repository/managing-alerts-from-secret-scanning)."
+Repository administrators and organization owners can grant users and team access to {% data variables.product.prodname_secret_scanning %} alerts. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-security-alerts)."
+
{% data variables.product.product_name %} currently scans private repositories for secrets issued by the following service providers.
- Adafruit
diff --git a/translations/ko-KR/content/github/administering-a-repository/classifying-your-repository-with-topics.md b/translations/ko-KR/content/github/administering-a-repository/classifying-your-repository-with-topics.md
index d4f1cf411bf6..43b1fa092c15 100644
--- a/translations/ko-KR/content/github/administering-a-repository/classifying-your-repository-with-topics.md
+++ b/translations/ko-KR/content/github/administering-a-repository/classifying-your-repository-with-topics.md
@@ -22,7 +22,7 @@ To browse the most used topics, go to https://github.com/topics/.
Repository admins can add any topics they'd like to a repository. Helpful topics to classify a repository include the repository's intended purpose, subject area, community, or language.{% if currentVersion == "free-pro-team@latest" %} Additionally, {% data variables.product.product_name %} analyzes public repository content and generates suggested topics that repository admins can accept or reject. Private repository content is not analyzed and does not receive topic suggestions.{% endif %}
-Public and private repositories can have topics, although you will only see private repositories that you have access to in topic search results.
+{% if currentVersion == "github-ae@latest" %}Internal {% else %}Public, internal, {% endif %}and private repositories can have topics, although you will only see private repositories that you have access to in topic search results.
You can search for repositories that are associated with a particular topic. For more information, see "[Searching for repositories](/articles/searching-for-repositories#search-by-topic)." You can also search for a list of topics on {% data variables.product.product_name %}. For more information, see "[Searching topics](/articles/searching-topics)."
diff --git a/translations/ko-KR/content/github/administering-a-repository/customizing-your-repositorys-social-media-preview.md b/translations/ko-KR/content/github/administering-a-repository/customizing-your-repositorys-social-media-preview.md
index 3c61f2eb0ed4..10a7cc56547a 100644
--- a/translations/ko-KR/content/github/administering-a-repository/customizing-your-repositorys-social-media-preview.md
+++ b/translations/ko-KR/content/github/administering-a-repository/customizing-your-repositorys-social-media-preview.md
@@ -11,7 +11,7 @@ versions:
Until you add an image, repository links expand to show basic information about the repository and the owner's avatar. Adding an image to your repository can help identify your project across various social platforms.
-You can upload an image to a private repository, but your image can only be shared from a public repository.
+{% if currentVersion != "github-ae@latest" %}You can upload an image to a private repository, but your image can only be shared from a public repository.{% endif %}
{% tip %}
Tip: Your image should be a PNG, JPG, or GIF file under 1 MB in size. For the best quality rendering, we recommend keeping the image at 640 by 320 pixels.
diff --git a/translations/ko-KR/content/github/administering-a-repository/deleting-a-repository.md b/translations/ko-KR/content/github/administering-a-repository/deleting-a-repository.md
index 95bf02446acb..df9e987dc69a 100644
--- a/translations/ko-KR/content/github/administering-a-repository/deleting-a-repository.md
+++ b/translations/ko-KR/content/github/administering-a-repository/deleting-a-repository.md
@@ -13,17 +13,16 @@ versions:
{% data reusables.organizations.owners-and-admins-can %} delete an organization repository. If **Allow members to delete or transfer repositories for this organization** has been disabled, only organization owners can delete organization repositories. {% data reusables.organizations.new-repo-permissions-more-info %}
-{% if currentVersion == "free-pro-team@latest" %}
+{% if currentVersion != "github-ae@latest" %}Deleting a public repository will not delete any forks of the repository.{% endif %}
+
{% warning %}
-**Warning**: Deleting a repository will **permanently** delete release attachments and team permissions. This action **cannot** be undone.
+**Warnings**:
-{% endwarning %}
-{% endif %}
+- Deleting a repository will **permanently** delete release attachments and team permissions. This action **cannot** be undone.
+- Deleting a private {% if currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" %}or internal {% endif %}repository will delete all forks of the repository.
-Please also keep in mind that:
-- Deleting a private repository will delete all of its forks.
-- Deleting a public repository will not delete its forks.
+{% endwarning %}
{% if currentVersion == "free-pro-team@latest" %}
You can restore some deleted repositories within 90 days. For more information, see "[Restoring a deleted repository](/articles/restoring-a-deleted-repository)."
diff --git a/translations/ko-KR/content/github/administering-a-repository/enabling-anonymous-git-read-access-for-a-repository.md b/translations/ko-KR/content/github/administering-a-repository/enabling-anonymous-git-read-access-for-a-repository.md
index 57550fe89a8b..5c740bfa0138 100644
--- a/translations/ko-KR/content/github/administering-a-repository/enabling-anonymous-git-read-access-for-a-repository.md
+++ b/translations/ko-KR/content/github/administering-a-repository/enabling-anonymous-git-read-access-for-a-repository.md
@@ -5,7 +5,6 @@ redirect_from:
- /articles/enabling-anonymous-git-read-access-for-a-repository
versions:
enterprise-server: '*'
- github-ae: '*'
---
Repository administrators can change the anonymous Git read access setting for a specific repository if:
diff --git a/translations/ko-KR/content/github/administering-a-repository/managing-repository-settings.md b/translations/ko-KR/content/github/administering-a-repository/managing-repository-settings.md
index 240e40b6ede2..fe7f46cdc3ec 100644
--- a/translations/ko-KR/content/github/administering-a-repository/managing-repository-settings.md
+++ b/translations/ko-KR/content/github/administering-a-repository/managing-repository-settings.md
@@ -1,6 +1,6 @@
---
title: Managing repository settings
-intro: 'Repository administrators and organization owners can change several settings, including the names and ownership of a repository and the public or private visibility of a repository. They can also delete a repository.'
+intro: 'Repository administrators and organization owners can change settings for a repository, like the name, ownership, and visibility, or delete the repository.'
mapTopic: true
redirect_from:
- /articles/managing-repository-settings
diff --git a/translations/ko-KR/content/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository.md b/translations/ko-KR/content/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository.md
index 31b5950b8bd7..a85aa34def2c 100644
--- a/translations/ko-KR/content/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository.md
+++ b/translations/ko-KR/content/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository.md
@@ -22,28 +22,28 @@ versions:
{% data reusables.repositories.navigate-to-security-and-analysis %}
4. Under "Configure security and analysis features", to the right of the feature, click **Disable** or **Enable**. 
-### Granting access to {% data variables.product.prodname_dependabot_alerts %}
+### Granting access to security alerts
-After you enable {% data variables.product.prodname_dependabot_alerts %} for a repository in an organization, organization owners and repository administrators can view the alerts by default. You can give additional teams and people access to the alerts for a repository.
+After you enable {% data variables.product.prodname_dependabot %} or {% data variables.product.prodname_secret_scanning %} alerts for a repository in an organization, organization owners and repository administrators can view the alerts by default. You can give additional teams and people access to the alerts for a repository.
{% note %}
-Organization owners and repository administrators can only grant access to view {% data variables.product.prodname_dependabot_alerts %} to people or teams who have write access to the repo.
+Organization owners and repository administrators can only grant access to view security alerts, such as {% data variables.product.prodname_dependabot %} and {% data variables.product.prodname_secret_scanning %} alerts, to people or teams who have write access to the repo.
{% endnote %}
{% data reusables.repositories.navigate-to-repo %}
{% data reusables.repositories.sidebar-settings %}
{% data reusables.repositories.navigate-to-security-and-analysis %}
-4. Under "Dependabot alerts", in the search field, start typing the name of the person or team you'd like to find, then click a name in the list of matches. 
-5. Click **Save changes**. 
+4. Under "Access to alerts", in the search field, start typing the name of the person or team you'd like to find, then click a name in the list of matches. 
+5. Click **Save changes**. 
-### Removing access to {% data variables.product.prodname_dependabot_alerts %}
+### Removing access to security alerts
{% data reusables.repositories.navigate-to-repo %}
{% data reusables.repositories.sidebar-settings %}
{% data reusables.repositories.navigate-to-security-and-analysis %}
-4. Under "Dependabot alerts", to the right of the person or team whose access you'd like to remove, click {% octicon "x" aria-label="X symbol" %}. 
+4. Under "Access to alerts", to the right of the person or team whose access you'd like to remove, click {% octicon "x" aria-label="X symbol" %}. 
### 더 읽을거리
diff --git a/translations/ko-KR/content/github/administering-a-repository/setting-repository-visibility.md b/translations/ko-KR/content/github/administering-a-repository/setting-repository-visibility.md
index a7179ff8feac..e3422e7084f3 100644
--- a/translations/ko-KR/content/github/administering-a-repository/setting-repository-visibility.md
+++ b/translations/ko-KR/content/github/administering-a-repository/setting-repository-visibility.md
@@ -19,17 +19,36 @@ Organization owners can restrict the ability to change repository visibility to
We recommend reviewing the following caveats before you change the visibility of a repository.
#### Making a repository private
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+* {% data variables.product.product_name %} will detach public forks of the public repository and put them into a new network. Public forks are not made private.{% endif %}
+* If you change a repository's visibility from internal to private, {% data variables.product.prodname_dotcom %} will remove forks that belong to any user without access to the newly private repository. {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}The visibility of any forks will also change to private.{% elsif currentVersion == "github-ae@latest" %}If the internal repository has any forks, the visibility of the forks is already private.{% endif %} For more information, see "[What happens to forks when a repository is deleted or changes visibility?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility)"{% if currentVersion == "free-pro-team@latest" %}
+* If you're using {% data variables.product.prodname_free_user %} for user accounts or organizations, some features won't be available in the repository after you change the visibility to private. {% data reusables.gated-features.more-info %}{% endif %}
+* Any published {% data variables.product.prodname_pages %} site will be automatically unpublished.{% if currentVersion == "free-pro-team@latest" %} If you added a custom domain to the {% data variables.product.prodname_pages %} site, you should remove or update your DNS records before making the repository private, to avoid the risk of a domain takeover. For more information, see "[Managing a custom domain for your {% data variables.product.prodname_pages %} site](/articles/managing-a-custom-domain-for-your-github-pages-site)."{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+* {% data variables.product.prodname_dotcom %} will no longer included the repository in the {% data variables.product.prodname_archive %}. For more information, see "[About archiving content and data on {% data variables.product.prodname_dotcom %}](/github/creating-cloning-and-archiving-repositories/about-archiving-content-and-data-on-github#about-the-github-archive-program)."{% endif %}{% if enterpriseServerVersions contains currentVersion %}
+* Anonymous Git read access is no longer available. For more information, see "[Enabling anonymous Git read access for a repository](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)."{% endif %}
- * {% data variables.product.prodname_dotcom %} will detach public forks of the public repository and put them into a new network. Public forks are not made private. {% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %}If you change a repository's visibility from internal to private, {% data variables.product.prodname_dotcom %} will remove forks that belong to any user without access to the newly private repository.{% endif %} For more information, see "[What happens to forks when a repository is deleted or changes visibility?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility#changing-a-public-repository-to-a-private-repository)"
- {% if currentVersion == "free-pro-team@latest" %}* If you're using {% data variables.product.prodname_free_user %} for user accounts or organizations, some features won't be available in the repository after you change the visibility to private. {% data reusables.gated-features.more-info %}
- * Any published {% data variables.product.prodname_pages %} site will be automatically unpublished. If you added a custom domain to the {% data variables.product.prodname_pages %} site, you should remove or update your DNS records before making the repository private, to avoid the risk of a domain takeover. For more information, see "[Managing a custom domain for your {% data variables.product.prodname_pages %} site](/articles/managing-a-custom-domain-for-your-github-pages-site)."
- * {% data variables.product.prodname_dotcom %} will no longer included the repository in the {% data variables.product.prodname_archive %}. For more information, see "[About archiving content and data on {% data variables.product.prodname_dotcom %}](/github/creating-cloning-and-archiving-repositories/about-archiving-content-and-data-on-github#about-the-github-archive-program)."{% endif %}
- {% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}* Anonymous Git read access is no longer available. For more information, see "[Enabling anonymous Git read access for a repository](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)."{% endif %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %}
+
+#### Making a repository internal
+
+{% note %}
+
+**Note:** {% data reusables.gated-features.internal-repos %}
+
+{% endnote %}
+
+* Any forks of the repository will remain in the repository network, and {% data variables.product.product_name %} maintains the relationship between the root repository and the fork. For more information, see "[What happens to forks when a repository is deleted or changes visibility?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility)"
+
+{% endif %}
+
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
#### Making a repository public
- * {% data variables.product.prodname_dotcom %} will detach private forks and turn them into a standalone private repository. For more information, see "[What happens to forks when a repository is deleted or changes visibility?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility#changing-a-private-repository-to-a-public-repository)"
- * If you're converting your private repository to a public repository as part of a move toward creating an open source project, see the [Open Source Guides](http://opensource.guide) for helpful tips and guidelines.{% if currentVersion == "free-pro-team@latest" %} You can also take a free course on managing an open source project with [{% data variables.product.prodname_learning %}]({% data variables.product.prodname_learning_link %}). Once your repository is public, you can also view your repository's community profile to see whether your project meets best practices for supporting contributors. For more information, see "[Viewing your community profile](/articles/viewing-your-community-profile)."{% endif %}
+* {% data variables.product.product_name %} will detach private forks and turn them into a standalone private repository. For more information, see "[What happens to forks when a repository is deleted or changes visibility?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility#changing-a-private-repository-to-a-public-repository)"{% if currentVersion == "free-pro-team@latest" %}
+* If you're converting your private repository to a public repository as part of a move toward creating an open source project, see the [Open Source Guides](http://opensource.guide) for helpful tips and guidelines. You can also take a free course on managing an open source project with [{% data variables.product.prodname_learning %}]({% data variables.product.prodname_learning_link %}). Once your repository is public, you can also view your repository's community profile to see whether your project meets best practices for supporting contributors. For more information, see "[Viewing your community profile](/articles/viewing-your-community-profile)."{% endif %}
+
+{% endif %}
{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
diff --git a/translations/ko-KR/content/github/authenticating-to-github/about-anonymized-image-urls.md b/translations/ko-KR/content/github/authenticating-to-github/about-anonymized-image-urls.md
index d0a0c03afa87..7484913676c1 100644
--- a/translations/ko-KR/content/github/authenticating-to-github/about-anonymized-image-urls.md
+++ b/translations/ko-KR/content/github/authenticating-to-github/about-anonymized-image-urls.md
@@ -8,7 +8,7 @@ versions:
free-pro-team: '*'
---
-To host your images, {% data variables.product.product_name %} uses the [open-source project Camo](https://github.com/atmos/camo). Camo generates an anonymous URL proxy for each image that starts with `https://camo.githubusercontent.com/` and hides your browser details and related information from other users.
+To host your images, {% data variables.product.product_name %} uses the [open-source project Camo](https://github.com/atmos/camo). Camo generates an anonymous URL proxy for each image which hides your browser details and related information from other users. The URL starts `https://.githubusercontent.com/`, with different subdomains depending on how you uploaded the image.
Anyone who receives your anonymized image URL, directly or indirectly, may view your image. To keep sensitive images private, restrict them to a private network or a server that requires authentication instead of using Camo.
diff --git a/translations/ko-KR/content/github/authenticating-to-github/adding-a-new-ssh-key-to-your-github-account.md b/translations/ko-KR/content/github/authenticating-to-github/adding-a-new-ssh-key-to-your-github-account.md
index e46804bc00e7..bb6a20540839 100644
--- a/translations/ko-KR/content/github/authenticating-to-github/adding-a-new-ssh-key-to-your-github-account.md
+++ b/translations/ko-KR/content/github/authenticating-to-github/adding-a-new-ssh-key-to-your-github-account.md
@@ -24,8 +24,8 @@ After adding a new SSH key to your {% data variables.product.product_name %} acc
If your SSH key file has a different name than the example code, modify the filename to match your current setup. When copying your key, don't add any newlines or whitespace.
```shell
- $ pbcopy < ~/.ssh/id_rsa.pub
- # Copies the contents of the id_rsa.pub file to your clipboard
+ $ pbcopy < ~/.ssh/id_ed25519.pub
+ # Copies the contents of the id_ed25519.pub file to your clipboard
```
{% tip %}
@@ -51,8 +51,8 @@ After adding a new SSH key to your {% data variables.product.product_name %} acc
If your SSH key file has a different name than the example code, modify the filename to match your current setup. When copying your key, don't add any newlines or whitespace.
```shell
- $ clip < ~/.ssh/id_rsa.pub
- # Copies the contents of the id_rsa.pub file to your clipboard
+ $ clip < ~/.ssh/id_ed25519.pub
+ # Copies the contents of the id_ed25519.pub file to your clipboard
```
{% tip %}
@@ -81,8 +81,8 @@ After adding a new SSH key to your {% data variables.product.product_name %} acc
$ sudo apt-get install xclip
# Downloads and installs xclip. If you don't have `apt-get`, you might need to use another installer (like `yum`)
- $ xclip -selection clipboard < ~/.ssh/id_rsa.pub
- # Copies the contents of the id_rsa.pub file to your clipboard
+ $ xclip -selection clipboard < ~/.ssh/id_ed25519.pub
+ # Copies the contents of the id_ed25519.pub file to your clipboard
```
{% tip %}
diff --git a/translations/ko-KR/content/github/authenticating-to-github/generating-a-new-ssh-key-and-adding-it-to-the-ssh-agent.md b/translations/ko-KR/content/github/authenticating-to-github/generating-a-new-ssh-key-and-adding-it-to-the-ssh-agent.md
index b19fc462f282..3fac8ec0bb95 100644
--- a/translations/ko-KR/content/github/authenticating-to-github/generating-a-new-ssh-key-and-adding-it-to-the-ssh-agent.md
+++ b/translations/ko-KR/content/github/authenticating-to-github/generating-a-new-ssh-key-and-adding-it-to-the-ssh-agent.md
@@ -98,13 +98,19 @@ Before adding a new SSH key to the ssh-agent to manage your keys, you should hav
IdentityFile ~/.ssh/id_ed25519
```
+ {% note %}
+
+ **Note:** If you chose not to add a passphrase to your key, you should omit the `UseKeychain` line.
+
+ {% endnote %}
+
3. Add your SSH private key to the ssh-agent and store your passphrase in the keychain. {% data reusables.ssh.add-ssh-key-to-ssh-agent %}
```shell
$ ssh-add -K ~/.ssh/id_ed25519
```
{% note %}
- **Note:** The `-K` option is Apple's standard version of `ssh-add`, which stores the passphrase in your keychain for you when you add an ssh key to the ssh-agent.
+ **Note:** The `-K` option is Apple's standard version of `ssh-add`, which stores the passphrase in your keychain for you when you add an ssh key to the ssh-agent. If you chose not to add a passphrase to your key, run the command without the `-K` option.
If you don't have Apple's standard version installed, you may receive an error. For more information on resolving this error, see "[Error: ssh-add: illegal option -- K](/articles/error-ssh-add-illegal-option-k)."
diff --git a/translations/ko-KR/content/github/authenticating-to-github/reviewing-your-security-log.md b/translations/ko-KR/content/github/authenticating-to-github/reviewing-your-security-log.md
index e1ba9b840db5..aa13e68ecd96 100644
--- a/translations/ko-KR/content/github/authenticating-to-github/reviewing-your-security-log.md
+++ b/translations/ko-KR/content/github/authenticating-to-github/reviewing-your-security-log.md
@@ -1,6 +1,7 @@
---
title: Reviewing your security log
intro: You can review the security log for your user account to better understand actions you've performed and actions others have performed that involve you.
+miniTocMaxHeadingLevel: 4
redirect_from:
- /articles/reviewing-your-security-log
versions:
@@ -30,216 +31,222 @@ The security log lists all actions performed within the last 90 days{% if curren
#### Search based on the action performed
{% else %}
### Understanding events in your security log
+{% endif %}
+
+The events listed in your security log are triggered by your actions. Actions are grouped into the following categories:
+
+| Category name | 설명 |
+| -------------------------------------------------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |{% if currentVersion == "free-pro-team@latest" %}
+| [`account_recovery_token`](#account_recovery_token-category-actions) | Contains all activities related to [adding a recovery token](/articles/configuring-two-factor-authentication-recovery-methods). |
+| [`결제`](#billing-category-actions) | Contains all activities related to your billing information. |
+| [`marketplace_agreement_signature`](#marketplace_agreement_signature-category-actions) | Contains all activities related to signing the {% data variables.product.prodname_marketplace %} Developer Agreement. |
+| [`marketplace_listing`](#marketplace_listing-category-actions) | Contains all activities related to listing apps in {% data variables.product.prodname_marketplace %}.{% endif %}
+| [`oauth_access`](#oauth_access-category-actions) | Contains all activities related to [{% data variables.product.prodname_oauth_app %}s](/articles/authorizing-oauth-apps) you've connected with.{% if currentVersion == "free-pro-team@latest" %}
+| [`payment_method`](#payment_method-category-actions) | Contains all activities related to paying for your {% data variables.product.prodname_dotcom %} subscription.{% endif %}
+| [`profile_picture`](#profile_picture-category-actions) | Contains all activities related to your profile picture. |
+| [`프로젝트`](#project-category-actions) | Contains all activities related to project boards. |
+| [`public_key`](#public_key-category-actions) | Contains all activities related to [your public SSH keys](/articles/adding-a-new-ssh-key-to-your-github-account). |
+| [`repo`](#repo-category-actions) | Contains all activities related to the repositories you own.{% if currentVersion == "free-pro-team@latest" %}
+| [`sponsors`](#sponsors-category-actions) | Contains all events related to {% data variables.product.prodname_sponsors %} and sponsor buttons (see "[About {% data variables.product.prodname_sponsors %}](/articles/about-github-sponsors)" and "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)"){% endif %}{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
+| [`팀`](#team-category-actions) | Contains all activities related to teams you are a part of.{% endif %}{% if currentVersion != "github-ae@latest" %}
+| [`two_factor_authentication`](#two_factor_authentication-category-actions) | Contains all activities related to [two-factor authentication](/articles/securing-your-account-with-two-factor-authentication-2fa).{% endif %}
+| [`사용자`](#user-category-actions) | Contains all activities related to your account. |
+
+{% if currentVersion == "free-pro-team@latest" %}
+
+### Exporting your security log
+
+{% data reusables.audit_log.export-log %}
+{% data reusables.audit_log.exported-log-keys-and-values %}
-Actions listed in your security log are grouped within the following categories: |{% endif %}
-| Category Name | 설명 |
-| ------------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |{% if currentVersion == "free-pro-team@latest" %}
-| `account_recovery_token` | Contains all activities related to [adding a recovery token](/articles/configuring-two-factor-authentication-recovery-methods). |
-| `결제` | Contains all activities related to your billing information. |
-| `marketplace_agreement_signature` | Contains all activities related to signing the {% data variables.product.prodname_marketplace %} Developer Agreement. |
-| `marketplace_listing` | Contains all activities related to listing apps in {% data variables.product.prodname_marketplace %}.{% endif %}
-| `oauth_access` | Contains all activities related to [{% data variables.product.prodname_oauth_app %}s](/articles/authorizing-oauth-apps) you've connected with.{% if currentVersion == "free-pro-team@latest" %}
-| `payment_method` | Contains all activities related to paying for your {% data variables.product.prodname_dotcom %} subscription.{% endif %}
-| `profile_picture` | Contains all activities related to your profile picture. |
-| `프로젝트` | Contains all activities related to project boards. |
-| `public_key` | Contains all activities related to [your public SSH keys](/articles/adding-a-new-ssh-key-to-your-github-account). |
-| `repo` | Contains all activities related to the repositories you own.{% if currentVersion == "free-pro-team@latest" %}
-| `sponsors` | Contains all events related to {% data variables.product.prodname_sponsors %} and sponsor buttons (see "[About {% data variables.product.prodname_sponsors %}](/articles/about-github-sponsors)" and "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)"){% endif %}{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-| `팀` | Contains all activities related to teams you are a part of.{% endif %}{% if currentVersion != "github-ae@latest" %}
-| `two_factor_authentication` | Contains all activities related to [two-factor authentication](/articles/securing-your-account-with-two-factor-authentication-2fa).{% endif %}
-| `사용자` | Contains all activities related to your account. |
-
-A description of the events within these categories is listed below.
+{% endif %}
+
+### Security log actions
+
+An overview of some of the most common actions that are recorded as events in the security log.
{% if currentVersion == "free-pro-team@latest" %}
-#### The `account_recovery_token` category
+#### `account_recovery_token` category actions
-| 동작 | 설명 |
-| ------------- | ----------------------------------------------------------------------------------------------------------------------------------------------- |
-| confirm | Triggered when you successfully [store a new token with a recovery provider](/articles/configuring-two-factor-authentication-recovery-methods). |
-| recover | Triggered when you successfully [redeem an account recovery token](/articles/recovering-your-account-if-you-lose-your-2fa-credentials). |
-| recover_error | Triggered when a token is used but {% data variables.product.prodname_dotcom %} is not able to validate it. |
+| 동작 | 설명 |
+| --------------- | ----------------------------------------------------------------------------------------------------------------------------------------------- |
+| `confirm` | Triggered when you successfully [store a new token with a recovery provider](/articles/configuring-two-factor-authentication-recovery-methods). |
+| `recover` | Triggered when you successfully [redeem an account recovery token](/articles/recovering-your-account-if-you-lose-your-2fa-credentials). |
+| `recover_error` | Triggered when a token is used but {% data variables.product.prodname_dotcom %} is not able to validate it. |
-#### The `billing` category
+#### `billing` category actions
| 동작 | 설명 |
| --------------------- | --------------------------------------------------------------------------------------------------------------------------------------- |
-| change_billing_type | Triggered when you [change how you pay](/articles/adding-or-editing-a-payment-method) for {% data variables.product.prodname_dotcom %}. |
-| change_email | Triggered when you [change your email address](/articles/changing-your-primary-email-address). |
+| `change_billing_type` | Triggered when you [change how you pay](/articles/adding-or-editing-a-payment-method) for {% data variables.product.prodname_dotcom %}. |
+| `change_email` | Triggered when you [change your email address](/articles/changing-your-primary-email-address). |
-#### The `marketplace_agreement_signature` category
+#### `marketplace_agreement_signature` category actions
-| 동작 | 설명 |
-| ------ | -------------------------------------------------------------------------------------------------- |
-| create | Triggered when you sign the {% data variables.product.prodname_marketplace %} Developer Agreement. |
+| 동작 | 설명 |
+| -------- | -------------------------------------------------------------------------------------------------- |
+| `create` | Triggered when you sign the {% data variables.product.prodname_marketplace %} Developer Agreement. |
-#### The `marketplace_listing` category
+#### `marketplace_listing` category actions
-| 동작 | 설명 |
-| ------- | --------------------------------------------------------------------------------------------------------------- |
-| 승인 | Triggered when your listing is approved for inclusion in {% data variables.product.prodname_marketplace %}. |
-| create | Triggered when you create a listing for your app in {% data variables.product.prodname_marketplace %}. |
-| delist | Triggered when your listing is removed from {% data variables.product.prodname_marketplace %}. |
-| redraft | Triggered when your listing is sent back to draft state. |
-| reject | Triggered when your listing is not accepted for inclusion in {% data variables.product.prodname_marketplace %}. |
+| 동작 | 설명 |
+| --------- | --------------------------------------------------------------------------------------------------------------- |
+| `승인` | Triggered when your listing is approved for inclusion in {% data variables.product.prodname_marketplace %}. |
+| `create` | Triggered when you create a listing for your app in {% data variables.product.prodname_marketplace %}. |
+| `delist` | Triggered when your listing is removed from {% data variables.product.prodname_marketplace %}. |
+| `redraft` | Triggered when your listing is sent back to draft state. |
+| `reject` | Triggered when your listing is not accepted for inclusion in {% data variables.product.prodname_marketplace %}. |
{% endif %}
-#### The `oauth_access` category
+#### `oauth_access` category actions
-| 동작 | 설명 |
-| ------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------ |
-| create | Triggered when you [grant access to an {% data variables.product.prodname_oauth_app %}](/articles/authorizing-oauth-apps). |
-| destroy | Triggered when you [revoke an {% data variables.product.prodname_oauth_app %}'s access to your account](/articles/reviewing-your-authorized-integrations). |
+| 동작 | 설명 |
+| --------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| `create` | Triggered when you [grant access to an {% data variables.product.prodname_oauth_app %}](/articles/authorizing-oauth-apps). |
+| `destroy` | Triggered when you [revoke an {% data variables.product.prodname_oauth_app %}'s access to your account](/articles/reviewing-your-authorized-integrations). |
{% if currentVersion == "free-pro-team@latest" %}
-#### The `payment_method` category
+#### `payment_method` category actions
-| 동작 | 설명 |
-| ------ | ------------------------------------------------------------------------------------------ |
-| clear | Triggered when [a payment method](/articles/removing-a-payment-method) on file is removed. |
-| create | Triggered when a new payment method is added, such as a new credit card or PayPal account. |
-| 업데이트 | Triggered when an existing payment method is updated. |
+| 동작 | 설명 |
+| -------- | ------------------------------------------------------------------------------------------ |
+| `clear` | Triggered when [a payment method](/articles/removing-a-payment-method) on file is removed. |
+| `create` | Triggered when a new payment method is added, such as a new credit card or PayPal account. |
+| `업데이트` | Triggered when an existing payment method is updated. |
{% endif %}
-#### The `profile_picture` category
+#### `profile_picture` category actions
-| 동작 | 설명 |
-| ---- | ------------------------------------------------------------------------------------------------- |
-| 업데이트 | Triggered when you [set or update your profile picture](/articles/setting-your-profile-picture/). |
+| 동작 | 설명 |
+| ------ | ------------------------------------------------------------------------------------------------- |
+| `업데이트` | Triggered when you [set or update your profile picture](/articles/setting-your-profile-picture/). |
-#### The `project` category
+#### `project` category actions
| 동작 | 설명 |
| ------------------------ | ------------------------------------------------------------------------------------------------------------------------- |
+| `액세스` | Triggered when a project board's visibility is changed. |
| `create` | Triggered when a project board is created. |
| `rename` | Triggered when a project board is renamed. |
| `업데이트` | Triggered when a project board is updated. |
| `delete` | Triggered when a project board is deleted. |
| `link` | Triggered when a repository is linked to a project board. |
| `unlink` | Triggered when a repository is unlinked from a project board. |
-| `project.access` | Triggered when a project board's visibility is changed. |
| `update_user_permission` | Triggered when an outside collaborator is added to or removed from a project board or has their permission level changed. |
-#### The `public_key` category
+#### `public_key` category actions
-| 동작 | 설명 |
-| ------ | --------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| create | Triggered when you [add a new public SSH key to your {% data variables.product.product_name %} account](/articles/adding-a-new-ssh-key-to-your-github-account). |
-| delete | Triggered when you [remove a public SSH key to your {% data variables.product.product_name %} account](/articles/reviewing-your-ssh-keys). |
+| 동작 | 설명 |
+| -------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `create` | Triggered when you [add a new public SSH key to your {% data variables.product.product_name %} account](/articles/adding-a-new-ssh-key-to-your-github-account). |
+| `delete` | Triggered when you [remove a public SSH key to your {% data variables.product.product_name %} account](/articles/reviewing-your-ssh-keys). |
-#### The `repo` category
+#### `repo` category actions
| 동작 | 설명 |
| ------------------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| 액세스 | Triggered when you a repository you own is [switched from "private" to "public"](/articles/making-a-private-repository-public) (or vice versa). |
-| add_member | Triggered when a {% data variables.product.product_name %} user is {% if currentVersion == "free-pro-team@latest" %}[invited to have collaboration access](/articles/inviting-collaborators-to-a-personal-repository){% else %}[given collaboration access](/articles/inviting-collaborators-to-a-personal-repository){% endif %} to a repository. |
-| add_topic | Triggered when a repository owner [adds a topic](/articles/classifying-your-repository-with-topics) to a repository. |
-| archived | Triggered when a repository owner [archives a repository](/articles/about-archiving-repositories).{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-| config.disable_anonymous_git_access | Triggered when [anonymous Git read access is disabled](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository) in a public repository. |
-| config.enable_anonymous_git_access | Triggered when [anonymous Git read access is enabled](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository) in a public repository. |
-| config.lock_anonymous_git_access | Triggered when a repository's [anonymous Git read access setting is locked](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access). |
-| config.unlock_anonymous_git_access | Triggered when a repository's [anonymous Git read access setting is unlocked](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access).{% endif %}
-| create | Triggered when [a new repository is created](/articles/creating-a-new-repository). |
-| destroy | Triggered when [a repository is deleted](/articles/deleting-a-repository).{% if currentVersion == "free-pro-team@latest" %}
-| 비활성화 | Triggered when a repository is disabled (e.g., for [insufficient funds](/articles/unlocking-a-locked-account)).{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-| 활성화 | Triggered when a repository is re-enabled.{% endif %}
-| remove_member | Triggered when a {% data variables.product.product_name %} user is [removed from a repository as a collaborator](/articles/removing-a-collaborator-from-a-personal-repository). |
-| remove_topic | Triggered when a repository owner removes a topic from a repository. |
-| rename | Triggered when [a repository is renamed](/articles/renaming-a-repository). |
-| 전송 | Triggered when [a repository is transferred](/articles/how-to-transfer-a-repository). |
-| transfer_start | Triggered when a repository transfer is about to occur. |
-| unarchived | Triggered when a repository owner unarchives a repository. |
+| `액세스` | Triggered when you a repository you own is [switched from "private" to "public"](/articles/making-a-private-repository-public) (or vice versa). |
+| `add_member` | Triggered when a {% data variables.product.product_name %} user is {% if currentVersion == "free-pro-team@latest" %}[invited to have collaboration access](/articles/inviting-collaborators-to-a-personal-repository){% else %}[given collaboration access](/articles/inviting-collaborators-to-a-personal-repository){% endif %} to a repository. |
+| `add_topic` | Triggered when a repository owner [adds a topic](/articles/classifying-your-repository-with-topics) to a repository. |
+| `archived` | Triggered when a repository owner [archives a repository](/articles/about-archiving-repositories).{% if enterpriseServerVersions contains currentVersion %}
+| `config.disable_anonymous_git_access` | Triggered when [anonymous Git read access is disabled](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository) in a public repository. |
+| `config.enable_anonymous_git_access` | Triggered when [anonymous Git read access is enabled](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository) in a public repository. |
+| `config.lock_anonymous_git_access` | Triggered when a repository's [anonymous Git read access setting is locked](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access). |
+| `config.unlock_anonymous_git_access` | Triggered when a repository's [anonymous Git read access setting is unlocked](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access).{% endif %}
+| `create` | Triggered when [a new repository is created](/articles/creating-a-new-repository). |
+| `destroy` | Triggered when [a repository is deleted](/articles/deleting-a-repository).{% if currentVersion == "free-pro-team@latest" %}
+| `비활성화` | Triggered when a repository is disabled (e.g., for [insufficient funds](/articles/unlocking-a-locked-account)).{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| `활성화` | Triggered when a repository is re-enabled.{% endif %}
+| `remove_member` | Triggered when a {% data variables.product.product_name %} user is [removed from a repository as a collaborator](/articles/removing-a-collaborator-from-a-personal-repository). |
+| `remove_topic` | Triggered when a repository owner removes a topic from a repository. |
+| `rename` | Triggered when [a repository is renamed](/articles/renaming-a-repository). |
+| `전송` | Triggered when [a repository is transferred](/articles/how-to-transfer-a-repository). |
+| `transfer_start` | Triggered when a repository transfer is about to occur. |
+| `unarchived` | Triggered when a repository owner unarchives a repository. |
{% if currentVersion == "free-pro-team@latest" %}
-#### The `sponsors` category
-
-| 동작 | 설명 |
-| ----------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
-| repo_funding_link_button_toggle | Triggered when you enable or disable a sponsor button in your repository (see "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)") |
-| repo_funding_links_file_action | Triggered when you change the FUNDING file in your repository (see "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)") |
-| sponsor_sponsorship_cancel | Triggered when you cancel a sponsorship (see "[Downgrading a sponsorship](/articles/downgrading-a-sponsorship)") |
-| sponsor_sponsorship_create | Triggered when you sponsor a developer (see "[Sponsoring an open source contributor](/github/supporting-the-open-source-community-with-github-sponsors/sponsoring-an-open-source-contributor#sponsoring-a-developer)") |
-| sponsor_sponsorship_preference_change | Triggered when you change whether you receive email updates from a sponsored developer (see "[Managing your sponsorship](/articles/managing-your-sponsorship)") |
-| sponsor_sponsorship_tier_change | Triggered when you upgrade or downgrade your sponsorship (see "[Upgrading a sponsorship](/articles/upgrading-a-sponsorship)" and "[Downgrading a sponsorship](/articles/downgrading-a-sponsorship)") |
-| sponsored_developer_approve | Triggered when your {% data variables.product.prodname_sponsors %} account is approved (see "[Setting up {% data variables.product.prodname_sponsors %} for your user account](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
-| sponsored_developer_create | Triggered when your {% data variables.product.prodname_sponsors %} account is created (see "[Setting up {% data variables.product.prodname_sponsors %} for your user account](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
-| sponsored_developer_profile_update | Triggered when you edit your sponsored developer profile (see "[Editing your profile details for {% data variables.product.prodname_sponsors %}](/github/supporting-the-open-source-community-with-github-sponsors/editing-your-profile-details-for-github-sponsors)") |
-| sponsored_developer_request_approval | Triggered when you submit your application for {% data variables.product.prodname_sponsors %} for approval (see "[Setting up {% data variables.product.prodname_sponsors %} for your user account](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
-| sponsored_developer_tier_description_update | Triggered when you change the description for a sponsorship tier (see "[Changing your sponsorship tiers](/articles/changing-your-sponsorship-tiers)") |
-| sponsored_developer_update_newsletter_send | Triggered when you send an email update to your sponsors (see "[Contacting your sponsors](/articles/contacting-your-sponsors)") |
-| waitlist_invite_sponsored_developer | Triggered when you are invited to join {% data variables.product.prodname_sponsors %} from the waitlist (see "[Setting up {% data variables.product.prodname_sponsors %} for your user account](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
-| waitlist_join | Triggered when you join the waitlist to become a sponsored developer (see "[Setting up {% data variables.product.prodname_sponsors %} for your user account](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
+#### `sponsors` category actions
+
+| 동작 | 설명 |
+| --------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| `repo_funding_link_button_toggle` | Triggered when you enable or disable a sponsor button in your repository (see "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)") |
+| `repo_funding_links_file_action` | Triggered when you change the FUNDING file in your repository (see "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)") |
+| `sponsor_sponsorship_cancel` | Triggered when you cancel a sponsorship (see "[Downgrading a sponsorship](/articles/downgrading-a-sponsorship)") |
+| `sponsor_sponsorship_create` | Triggered when you sponsor a developer (see "[Sponsoring an open source contributor](/github/supporting-the-open-source-community-with-github-sponsors/sponsoring-an-open-source-contributor#sponsoring-a-developer)") |
+| `sponsor_sponsorship_preference_change` | Triggered when you change whether you receive email updates from a sponsored developer (see "[Managing your sponsorship](/articles/managing-your-sponsorship)") |
+| `sponsor_sponsorship_tier_change` | Triggered when you upgrade or downgrade your sponsorship (see "[Upgrading a sponsorship](/articles/upgrading-a-sponsorship)" and "[Downgrading a sponsorship](/articles/downgrading-a-sponsorship)") |
+| `sponsored_developer_approve` | Triggered when your {% data variables.product.prodname_sponsors %} account is approved (see "[Setting up {% data variables.product.prodname_sponsors %} for your user account](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
+| `sponsored_developer_create` | Triggered when your {% data variables.product.prodname_sponsors %} account is created (see "[Setting up {% data variables.product.prodname_sponsors %} for your user account](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
+| `sponsored_developer_profile_update` | Triggered when you edit your sponsored developer profile (see "[Editing your profile details for {% data variables.product.prodname_sponsors %}](/github/supporting-the-open-source-community-with-github-sponsors/editing-your-profile-details-for-github-sponsors)") |
+| `sponsored_developer_request_approval` | Triggered when you submit your application for {% data variables.product.prodname_sponsors %} for approval (see "[Setting up {% data variables.product.prodname_sponsors %} for your user account](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
+| `sponsored_developer_tier_description_update` | Triggered when you change the description for a sponsorship tier (see "[Changing your sponsorship tiers](/articles/changing-your-sponsorship-tiers)") |
+| `sponsored_developer_update_newsletter_send` | Triggered when you send an email update to your sponsors (see "[Contacting your sponsors](/articles/contacting-your-sponsors)") |
+| `waitlist_invite_sponsored_developer` | Triggered when you are invited to join {% data variables.product.prodname_sponsors %} from the waitlist (see "[Setting up {% data variables.product.prodname_sponsors %} for your user account](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
+| `waitlist_join` | Triggered when you join the waitlist to become a sponsored developer (see "[Setting up {% data variables.product.prodname_sponsors %} for your user account](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
{% endif %}
{% if currentVersion == "free-pro-team@latest" %}
-#### The `successor_invitation` category
-
-| 동작 | 설명 |
-| ------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| accept | Triggered when you accept a succession invitation (see "[Maintaining ownership continuity of your user account's repositories](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
-| cancel | Triggered when you cancel a succession invitation (see "[Maintaining ownership continuity of your user account's repositories](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
-| create | Triggered when you create a succession invitation (see "[Maintaining ownership continuity of your user account's repositories](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
-| decline | Triggered when you decline a succession invitation (see "[Maintaining ownership continuity of your user account's repositories](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
-| revoke | Triggered when you revoke a succession invitation (see "[Maintaining ownership continuity of your user account's repositories](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
+#### `successor_invitation` category actions
+
+| 동작 | 설명 |
+| --------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `accept` | Triggered when you accept a succession invitation (see "[Maintaining ownership continuity of your user account's repositories](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
+| `cancel` | Triggered when you cancel a succession invitation (see "[Maintaining ownership continuity of your user account's repositories](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
+| `create` | Triggered when you create a succession invitation (see "[Maintaining ownership continuity of your user account's repositories](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
+| `decline` | Triggered when you decline a succession invitation (see "[Maintaining ownership continuity of your user account's repositories](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
+| `revoke` | Triggered when you revoke a succession invitation (see "[Maintaining ownership continuity of your user account's repositories](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
{% endif %}
{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-#### The `team` category
+#### `team` category actions
-| 동작 | 설명 |
-| ----------------- | --------------------------------------------------------------------------------------------------------------------------------------------- |
-| add_member | Triggered when a member of an organization you belong to [adds you to a team](/articles/adding-organization-members-to-a-team). |
-| add_repository | Triggered when a team you are a member of is given control of a repository. |
-| create | Triggered when a new team in an organization you belong to is created. |
-| destroy | Triggered when a team you are a member of is deleted from the organization. |
-| remove_member | Triggered when a member of an organization is [removed from a team](/articles/removing-organization-members-from-a-team) you are a member of. |
-| remove_repository | Triggered when a repository is no longer under a team's control. |
+| 동작 | 설명 |
+| ------------------- | --------------------------------------------------------------------------------------------------------------------------------------------- |
+| `add_member` | Triggered when a member of an organization you belong to [adds you to a team](/articles/adding-organization-members-to-a-team). |
+| `add_repository` | Triggered when a team you are a member of is given control of a repository. |
+| `create` | Triggered when a new team in an organization you belong to is created. |
+| `destroy` | Triggered when a team you are a member of is deleted from the organization. |
+| `remove_member` | Triggered when a member of an organization is [removed from a team](/articles/removing-organization-members-from-a-team) you are a member of. |
+| `remove_repository` | Triggered when a repository is no longer under a team's control. |
{% endif %}
{% if currentVersion != "github-ae@latest" %}
-#### The `two_factor_authentication` category
+#### `two_factor_authentication` category actions
-| 동작 | 설명 |
-| -------- | -------------------------------------------------------------------------------------------------------------------------- |
-| enabled | Triggered when [two-factor authentication](/articles/securing-your-account-with-two-factor-authentication-2fa) is enabled. |
-| disabled | Triggered when two-factor authentication is disabled. |
+| 동작 | 설명 |
+| ---------- | -------------------------------------------------------------------------------------------------------------------------- |
+| `enabled` | Triggered when [two-factor authentication](/articles/securing-your-account-with-two-factor-authentication-2fa) is enabled. |
+| `disabled` | Triggered when two-factor authentication is disabled. |
{% endif %}
-#### The `user` category
-
-| 동작 | 설명 |
-| ---------------------------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| add_email | Triggered when you {% if currentVersion != "github-ae@latest" %}[add a new email address](/articles/changing-your-primary-email-address){% else %}add a new email address{% endif %}. |
-| create | Triggered when you create a new user account. |
-| remove_email | Triggered when you remove an email address. |
-| rename | Triggered when you rename your account.{% if currentVersion != "github-ae@latest" %}
-| change_password | Triggered when you change your password. |
-| forgot_password | Triggered when you ask for [a password reset](/articles/how-can-i-reset-my-password).{% endif %}
-| login | Triggered when you log in to {% data variables.product.product_location %}. |
-| failed_login | Triggered when you failed to log in successfully.{% if currentVersion != "github-ae@latest" %}
-| two_factor_requested | Triggered when {% data variables.product.product_name %} asks you for [your two-factor authentication code](/articles/accessing-github-using-two-factor-authentication).{% endif %}
-| show_private_contributions_count | Triggered when you [publicize private contributions on your profile](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile). |
-| hide_private_contributions_count | Triggered when you [hide private contributions on your profile](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile).{% if currentVersion == "free-pro-team@latest" %}
-| report_content | Triggered when you [report an issue or pull request, or a comment on an issue, pull request, or commit](/articles/reporting-abuse-or-spam).{% endif %}
-
-#### The `user_status` category
-
-| 동작 | 설명 |
-| ------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| 업데이트 | Triggered when you set or change the status on your profile. For more information, see "[Setting a status](/articles/personalizing-your-profile/#setting-a-status)." |
-| destroy | Triggered when you clear the status on your profile. |
+#### `user` category actions
-{% if currentVersion == "free-pro-team@latest" %}
+| 동작 | 설명 |
+| ---------------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `add_email` | Triggered when you {% if currentVersion != "github-ae@latest" %}[add a new email address](/articles/changing-your-primary-email-address){% else %}add a new email address{% endif %}. |
+| `create` | Triggered when you create a new user account.{% if currentVersion != "github-ae@latest" %}
+| `change_password` | Triggered when you change your password. |
+| `forgot_password` | Triggered when you ask for [a password reset](/articles/how-can-i-reset-my-password).{% endif %}
+| `hide_private_contributions_count` | Triggered when you [hide private contributions on your profile](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile). |
+| `login` | Triggered when you log in to {% data variables.product.product_location %}. |
+| `failed_login` | Triggered when you failed to log in successfully. |
+| `remove_email` | Triggered when you remove an email address. |
+| `rename` | Triggered when you rename your account.{% if currentVersion == "free-pro-team@latest" %}
+| `report_content` | Triggered when you [report an issue or pull request, or a comment on an issue, pull request, or commit](/articles/reporting-abuse-or-spam).{% endif %}
+| `show_private_contributions_count` | Triggered when you [publicize private contributions on your profile](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile).{% if currentVersion != "github-ae@latest" %}
+| `two_factor_requested` | Triggered when {% data variables.product.product_name %} asks you for [your two-factor authentication code](/articles/accessing-github-using-two-factor-authentication).{% endif %}
-### Exporting your security log
+#### `user_status` category actions
+
+| 동작 | 설명 |
+| --------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `업데이트` | Triggered when you set or change the status on your profile. For more information, see "[Setting a status](/articles/personalizing-your-profile/#setting-a-status)." |
+| `destroy` | Triggered when you clear the status on your profile. |
-{% data reusables.audit_log.export-log %}
-{% data reusables.audit_log.exported-log-keys-and-values %}
-{% endif %}
diff --git a/translations/ko-KR/content/github/building-a-strong-community/about-issue-and-pull-request-templates.md b/translations/ko-KR/content/github/building-a-strong-community/about-issue-and-pull-request-templates.md
index c45a6e764fc6..8d8c0a7b0e5b 100644
--- a/translations/ko-KR/content/github/building-a-strong-community/about-issue-and-pull-request-templates.md
+++ b/translations/ko-KR/content/github/building-a-strong-community/about-issue-and-pull-request-templates.md
@@ -11,7 +11,11 @@ versions:
After you create issue and pull request templates in your repository, contributors can use the templates to open issues or describe the proposed changes in their pull requests according to the repository's contributing guidelines. For more information about adding contributing guidelines to a repository, see "[Setting guidelines for repository contributors](/articles/setting-guidelines-for-repository-contributors)."
-You can create default issue and pull request templates for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. For more information, see "[Creating a default community health file](/github/building-a-strong-community/creating-a-default-community-health-file)."
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+You can create default issue and pull request templates for your organization or user account. For more information, see "[Creating a default community health file](/github/building-a-strong-community/creating-a-default-community-health-file)."
+
+{% endif %}
### Issue templates
diff --git a/translations/ko-KR/content/github/building-a-strong-community/about-wikis.md b/translations/ko-KR/content/github/building-a-strong-community/about-wikis.md
index 9765903f050c..849560e91843 100644
--- a/translations/ko-KR/content/github/building-a-strong-community/about-wikis.md
+++ b/translations/ko-KR/content/github/building-a-strong-community/about-wikis.md
@@ -15,9 +15,9 @@ Every {% data variables.product.product_name %} repository comes equipped with a
With wikis, you can write content just like everywhere else on {% data variables.product.product_name %}. For more information, see "[Getting started with writing and formatting on {% data variables.product.prodname_dotcom %}](/articles/getting-started-with-writing-and-formatting-on-github)." We use [our open-source Markup library](https://github.com/github/markup) to convert different formats into HTML, so you can choose to write in Markdown or any other supported format.
-Wikis are available to the public in public repositories, and limited to people with access to the repository in private repositories. For more information, see "[Setting repository visibility](/articles/setting-repository-visibility)."
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}If you create a wiki in a public repository, the wiki is available to {% if enterpriseServerVersions contains currentVersion %}anyone with access to {% data variables.product.product_location %}{% else %}the public{% endif %}. {% endif %}If you create a wiki in an internal or private repository, {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}people{% elsif currentVersion == "github-ae@latest" %}enterprise members{% endif %} with access to the repository can also access the wiki. For more information, see "[Setting repository visibility](/articles/setting-repository-visibility)."
-You can edit wikis directly on {% data variables.product.product_name %}, or you can edit wiki files locally. By default, only people with write access to your repository can make changes to wikis, although you can allow everyone on {% data variables.product.product_name %} to contribute to a wiki in a public repository. For more information, see "[Changing access permissions for wikis](/articles/changing-access-permissions-for-wikis)".
+You can edit wikis directly on {% data variables.product.product_name %}, or you can edit wiki files locally. By default, only people with write access to your repository can make changes to wikis, although you can allow everyone on {% data variables.product.product_location %} to contribute to a wiki in {% if currentVersion == "github-ae@latest" %}an internal{% else %}a public{% endif %} repository. For more information, see "[Changing access permissions for wikis](/articles/changing-access-permissions-for-wikis)".
### 더 읽을거리
diff --git a/translations/ko-KR/content/github/building-a-strong-community/adding-a-license-to-a-repository.md b/translations/ko-KR/content/github/building-a-strong-community/adding-a-license-to-a-repository.md
index 57d811bfb560..4b158b6b2cac 100644
--- a/translations/ko-KR/content/github/building-a-strong-community/adding-a-license-to-a-repository.md
+++ b/translations/ko-KR/content/github/building-a-strong-community/adding-a-license-to-a-repository.md
@@ -6,7 +6,6 @@ redirect_from:
versions:
free-pro-team: '*'
enterprise-server: '*'
- github-ae: '*'
---
If you include a detectable license in your repository, people who visit your repository will see it at the top of the repository page. To read the entire license file, click the license name.
diff --git a/translations/ko-KR/content/github/building-a-strong-community/adding-support-resources-to-your-project.md b/translations/ko-KR/content/github/building-a-strong-community/adding-support-resources-to-your-project.md
index 7856e3860fd5..397caf7fcfe8 100644
--- a/translations/ko-KR/content/github/building-a-strong-community/adding-support-resources-to-your-project.md
+++ b/translations/ko-KR/content/github/building-a-strong-community/adding-support-resources-to-your-project.md
@@ -13,7 +13,11 @@ To direct people to specific support resources, you can add a SUPPORT file to yo

-You can create default support resources for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. For more information, see "[Creating a default community health file](/github/building-a-strong-community/creating-a-default-community-health-file)."
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+You can create default support resources for your organization or user account. For more information, see "[Creating a default community health file](/github/building-a-strong-community/creating-a-default-community-health-file)."
+
+{% endif %}
{% tip %}
diff --git a/translations/ko-KR/content/github/building-a-strong-community/configuring-issue-templates-for-your-repository.md b/translations/ko-KR/content/github/building-a-strong-community/configuring-issue-templates-for-your-repository.md
index 4062ff87fbeb..7b34ddcf8edc 100644
--- a/translations/ko-KR/content/github/building-a-strong-community/configuring-issue-templates-for-your-repository.md
+++ b/translations/ko-KR/content/github/building-a-strong-community/configuring-issue-templates-for-your-repository.md
@@ -10,10 +10,16 @@ versions:
github-ae: '*'
---
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
{% data reusables.repositories.default-issue-templates %}
+{% endif %}
+
{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %}
+
### Creating issue templates
+
{% endif %}
{% data reusables.repositories.navigate-to-repo %}
diff --git a/translations/ko-KR/content/github/building-a-strong-community/creating-a-default-community-health-file.md b/translations/ko-KR/content/github/building-a-strong-community/creating-a-default-community-health-file.md
index 1266f153157c..e12a3c4bc0eb 100644
--- a/translations/ko-KR/content/github/building-a-strong-community/creating-a-default-community-health-file.md
+++ b/translations/ko-KR/content/github/building-a-strong-community/creating-a-default-community-health-file.md
@@ -7,7 +7,6 @@ redirect_from:
versions:
free-pro-team: '*'
enterprise-server: '*'
- github-ae: '*'
---
### About default community health files
diff --git a/translations/ko-KR/content/github/building-a-strong-community/creating-a-pull-request-template-for-your-repository.md b/translations/ko-KR/content/github/building-a-strong-community/creating-a-pull-request-template-for-your-repository.md
index 0dd281d41f52..c89b6554defc 100644
--- a/translations/ko-KR/content/github/building-a-strong-community/creating-a-pull-request-template-for-your-repository.md
+++ b/translations/ko-KR/content/github/building-a-strong-community/creating-a-pull-request-template-for-your-repository.md
@@ -13,7 +13,11 @@ For more information, see "[About issue and pull request templates](/articles/ab
You can create a *PULL_REQUEST_TEMPLATE/* subdirectory in any of the supported folders to contain multiple pull request templates, and use the `template` query parameter to specify the template that will fill the pull request body. For more information, see "[About automation for issues and pull requests with query parameters](/articles/about-automation-for-issues-and-pull-requests-with-query-parameters)."
-You can create default pull request templates for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. For more information, see "[Creating a default community health file](/github/building-a-strong-community/creating-a-default-community-health-file)."
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+You can create default pull request templates for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. For more information, see "[Creating a default community health file](/github/building-a-strong-community/creating-a-default-community-health-file)."
+
+{% endif %}
### Adding a pull request template
diff --git a/translations/ko-KR/content/github/building-a-strong-community/locking-conversations.md b/translations/ko-KR/content/github/building-a-strong-community/locking-conversations.md
index c7980b882153..8014ecf22722 100644
--- a/translations/ko-KR/content/github/building-a-strong-community/locking-conversations.md
+++ b/translations/ko-KR/content/github/building-a-strong-community/locking-conversations.md
@@ -15,7 +15,7 @@ Locking a conversation creates a timeline event that is visible to anyone with r

-While a conversation is locked, only [people with write access](/articles/repository-permission-levels-for-an-organization/) and [repository owners and collaborators](/articles/permission-levels-for-a-user-account-repository/#collaborator-access-on-a-repository-owned-by-a-user-account) can add, hide, and delete comments.
+While a conversation is locked, only [people with write access](/articles/repository-permission-levels-for-an-organization/) and [repository owners and collaborators](/articles/permission-levels-for-a-user-account-repository/#collaborator-access-for-a-repository-owned-by-a-user-account) can add, hide, and delete comments.
To search for locked conversations in a repository that is not archived, you can use the search qualifiers `is:locked` and `archived:false`. Conversations are automatically locked in archived repositories. For more information, see "[Searching issues and pull requests](/articles/searching-issues-and-pull-requests#search-based-on-whether-a-conversation-is-locked)."
diff --git a/translations/ko-KR/content/github/building-a-strong-community/manually-creating-a-single-issue-template-for-your-repository.md b/translations/ko-KR/content/github/building-a-strong-community/manually-creating-a-single-issue-template-for-your-repository.md
index dbb20f73ad33..40f359a88a69 100644
--- a/translations/ko-KR/content/github/building-a-strong-community/manually-creating-a-single-issue-template-for-your-repository.md
+++ b/translations/ko-KR/content/github/building-a-strong-community/manually-creating-a-single-issue-template-for-your-repository.md
@@ -39,8 +39,12 @@ assignees: octocat
{% endif %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
{% data reusables.repositories.default-issue-templates %}
+{% endif %}
+
### Adding an issue template
{% data reusables.repositories.navigate-to-repo %}
diff --git a/translations/ko-KR/content/github/building-a-strong-community/setting-guidelines-for-repository-contributors.md b/translations/ko-KR/content/github/building-a-strong-community/setting-guidelines-for-repository-contributors.md
index 5267f7dd8d65..630e728697ae 100644
--- a/translations/ko-KR/content/github/building-a-strong-community/setting-guidelines-for-repository-contributors.md
+++ b/translations/ko-KR/content/github/building-a-strong-community/setting-guidelines-for-repository-contributors.md
@@ -20,7 +20,11 @@ For contributors, the guidelines help them verify that they're submitting well-f
For both owners and contributors, contribution guidelines save time and hassle caused by improperly created pull requests or issues that have to be rejected and re-submitted.
-You can create default contribution guidelines for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. For more information, see "[Creating a default community health file](/github/building-a-strong-community/creating-a-default-community-health-file)."
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+You can create default contribution guidelines for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. For more information, see "[Creating a default community health file](/github/building-a-strong-community/creating-a-default-community-health-file)."
+
+{% endif %}
{% tip %}
@@ -53,5 +57,5 @@ If you're stumped, here are some good examples of contribution guidelines:
### 더 읽을거리
- The Open Source Guides' section "[Starting an Open Source Project](https://opensource.guide/starting-a-project/)"{% if currentVersion == "free-pro-team@latest" %}
-- [{% data variables.product.prodname_learning %}]({% data variables.product.prodname_learning_link %}){% endif %}
-- "[Adding a license to a repository](/articles/adding-a-license-to-a-repository)"
+- [{% data variables.product.prodname_learning %}]({% data variables.product.prodname_learning_link %}){% endif %}{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- "[Adding a license to a repository](/articles/adding-a-license-to-a-repository)"{% endif %}
diff --git a/translations/ko-KR/content/github/collaborating-with-issues-and-pull-requests/about-branches.md b/translations/ko-KR/content/github/collaborating-with-issues-and-pull-requests/about-branches.md
index 07fa74edc692..ac8592ffee3e 100644
--- a/translations/ko-KR/content/github/collaborating-with-issues-and-pull-requests/about-branches.md
+++ b/translations/ko-KR/content/github/collaborating-with-issues-and-pull-requests/about-branches.md
@@ -23,7 +23,7 @@ You must have write access to a repository to create a branch, open a pull reque
### About the default branch
-{% data reusables.branches.new-repo-default-branch %} The default branch is the branch that {% data variables.product.prodname_dotcom %} displays when anyone visits your repository. The default branch is also the initial branch that Git checks out locally out when someone clones the repository. {% data reusables.branches.default-branch-automatically-base-branch %}
+{% data reusables.branches.new-repo-default-branch %} The default branch is the branch that {% data variables.product.prodname_dotcom %} displays when anyone visits your repository. The default branch is also the initial branch that Git checks out locally when someone clones the repository. {% data reusables.branches.default-branch-automatically-base-branch %}
By default, {% data variables.product.product_name %} names the default branch {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}`main`{% else %}`master`{% endif %} in any new repository.
@@ -75,7 +75,7 @@ When a branch is protected:
- If required pull request reviews are enabled on the branch, you won't be able to merge changes into the branch until all requirements in the pull request review policy have been met. For more information, see "[Merging a pull request](/articles/merging-a-pull-request)."
- If required review from a code owner is enabled on a branch, and a pull request modifies code that has an owner, a code owner must approve the pull request before it can be merged. For more information, see "[About code owners](/articles/about-code-owners)."
- If required commit signing is enabled on a branch, you won't be able to push any commits to the branch that are not signed and verified. For more information, see "[About commit signature verification](/articles/about-commit-signature-verification)" and "[About required commit signing](/articles/about-required-commit-signing)."{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
-- If you use {% data variables.product.prodname_dotcom %} 's conflict editor to fix conflicts for a pull request that you created from a protected branch, {% data variables.product.prodname_dotcom %} helps you to create an alternative branch for the pull request, so that your resolution of the conflicts can be merged. For more information, see "[Resolving a merge conflict on {% data variables.product.prodname_dotcom %}](/github/collaborating-with-issues-and-pull-requests/resolving-a-merge-conflict-on-github)."{% endif %}
+- If you use {% data variables.product.prodname_dotcom %}'s conflict editor to fix conflicts for a pull request that you created from a protected branch, {% data variables.product.prodname_dotcom %} helps you to create an alternative branch for the pull request, so that your resolution of the conflicts can be merged. For more information, see "[Resolving a merge conflict on {% data variables.product.prodname_dotcom %}](/github/collaborating-with-issues-and-pull-requests/resolving-a-merge-conflict-on-github)."{% endif %}
### 더 읽을거리
diff --git a/translations/ko-KR/content/github/collaborating-with-issues-and-pull-requests/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility.md b/translations/ko-KR/content/github/collaborating-with-issues-and-pull-requests/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility.md
index b6c200db082e..043241daffae 100644
--- a/translations/ko-KR/content/github/collaborating-with-issues-and-pull-requests/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility.md
+++ b/translations/ko-KR/content/github/collaborating-with-issues-and-pull-requests/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility.md
@@ -16,14 +16,20 @@ versions:
When you delete a private repository, all of its private forks are also deleted.
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
#### Deleting a public repository
When you delete a public repository, one of the existing public forks is chosen to be the new parent repository. All other repositories are forked off of this new parent and subsequent pull requests go to this new parent.
+{% endif %}
+
#### Private forks and permissions
{% data reusables.repositories.private_forks_inherit_permissions %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
#### Changing a public repository to a private repository
If a public repository is made private, its public forks are split off into a new network. As with deleting a public repository, one of the existing public forks is chosen to be the new parent repository and all other repositories are forked off of this new parent. Subsequent pull requests go to this new parent.
@@ -46,9 +52,30 @@ If a private repository is made public, each of its private forks is turned into
If a private repository is made public and then deleted, its private forks will continue to exist as standalone private repositories in separate networks.
+{% endif %}
+
+{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %}
+
+#### Changing the visibility of an internal repository
+
+{% note %}
+
+**Note:** {% data reusables.gated-features.internal-repos %}
+
+{% endnote %}
+
+If the policy for your enterprise permits forking, any fork of an internal repository will be private. If you change the visibility of an internal repository, any fork owned by an organization or user account will remain private.
+
+##### Deleting the internal repository
+
+If you change the visibility of an internal repository and then delete the repository, the forks will continue to exist in a separate network.
+
+{% endif %}
+
### 더 읽을거리
- "[Setting repository visibility](/articles/setting-repository-visibility)"
- "[About forks](/articles/about-forks)"
- "[Managing the forking policy for your repository](/github/administering-a-repository/managing-the-forking-policy-for-your-repository)"
- "[Managing the forking policy for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-the-forking-policy-for-your-organization)"
+- "{% if currentVersion == "free-pro-team@latest" %}[Enforcing repository management policies in your enterprise account](/github/setting-up-and-managing-your-enterprise/enforcing-repository-management-policies-in-your-enterprise-account#enforcing-a-policy-on-forking-private-or-internal-repositories){% else %}[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#enforcing-a-policy-on-forking-private-or-internal-repositories){% endif %}"
diff --git a/translations/ko-KR/content/github/creating-cloning-and-archiving-repositories/about-readmes.md b/translations/ko-KR/content/github/creating-cloning-and-archiving-repositories/about-readmes.md
index 5955da5726d5..9c8706d06090 100644
--- a/translations/ko-KR/content/github/creating-cloning-and-archiving-repositories/about-readmes.md
+++ b/translations/ko-KR/content/github/creating-cloning-and-archiving-repositories/about-readmes.md
@@ -11,7 +11,11 @@ versions:
github-ae: '*'
---
-A README file, along with {% if currentVersion == "free-pro-team@latest" %}a [repository license](/articles/licensing-a-repository), [contribution guidelines](/articles/setting-guidelines-for-repository-contributors), and a [code of conduct](/articles/adding-a-code-of-conduct-to-your-project){% else %}a [repository license](/articles/licensing-a-repository) and [contribution guidelines](/articles/setting-guidelines-for-repository-contributors){% endif %}, helps you communicate expectations for and manage contributions to your project.
+### About READMEs
+
+You can add a README file to a repository to communicate important information about your project. A README, along with a repository license{% if currentVersion == "free-pro-team@latest" %}, contribution guidelines, and a code of conduct{% elsif enterpriseServerVersions contains currentVersion %} and contribution guidelines{% endif %}, communicates expectations for your project and helps you manage contributions.
+
+For more information about providing guidelines for your project, see {% if currentVersion == "free-pro-team@latest" %}"[Adding a code of conduct to your project](/github/building-a-strong-community/adding-a-code-of-conduct-to-your-project)" and {% endif %}"[Setting up your project for healthy contributions](/github/building-a-strong-community/setting-up-your-project-for-healthy-contributions)."
A README is often the first item a visitor will see when visiting your repository. README files typically include information on:
- What the project does
@@ -26,8 +30,12 @@ If you put your README file in your repository's root, `docs`, or hidden `.githu
{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21"%}
+
{% data reusables.profile.profile-readme %}
+{% endif %}
+

{% endif %}
diff --git a/translations/ko-KR/content/github/creating-cloning-and-archiving-repositories/licensing-a-repository.md b/translations/ko-KR/content/github/creating-cloning-and-archiving-repositories/licensing-a-repository.md
index f6ccb4a676c2..6b51db17f0d6 100644
--- a/translations/ko-KR/content/github/creating-cloning-and-archiving-repositories/licensing-a-repository.md
+++ b/translations/ko-KR/content/github/creating-cloning-and-archiving-repositories/licensing-a-repository.md
@@ -7,7 +7,6 @@ redirect_from:
versions:
free-pro-team: '*'
enterprise-server: '*'
- github-ae: '*'
---
### Choosing the right license
@@ -18,7 +17,7 @@ You're under no obligation to choose a license. However, without a license, the
{% note %}
-**Note:** If you publish your source code in a public repository on GitHub, {% if currentVersion == "free-pro-team@latest" %}according to the [Terms of Service](/articles/github-terms-of-service), {% endif %}other GitHub users have the right to view and fork your repository within the GitHub site. If you have already created a public repository and no longer want users to have access to it, you can make your repository private. When you convert a public repository to a private repository, existing forks or local copies created by other users will still exist. For more information, see "[Making a public repository private](/articles/making-a-public-repository-private)."
+**Note:** If you publish your source code in a public repository on {% data variables.product.product_name %}, {% if currentVersion == "free-pro-team@latest" %}according to the [Terms of Service](/articles/github-terms-of-service), {% endif %}other users of {% data variables.product.product_location %} have the right to view and fork your repository. If you have already created a repository and no longer want users to have access to the repository, you can make the repository private. When you change the visibility of a repository to private, existing forks or local copies created by other users will still exist. For more information, see "[Setting repository visibility](/github/administering-a-repository/setting-repository-visibility)."
{% endnote %}
diff --git a/translations/ko-KR/content/github/developing-online-with-codespaces/using-codespaces-in-visual-studio.md b/translations/ko-KR/content/github/developing-online-with-codespaces/using-codespaces-in-visual-studio.md
index 9837bc2a7aea..5760feb54cb1 100644
--- a/translations/ko-KR/content/github/developing-online-with-codespaces/using-codespaces-in-visual-studio.md
+++ b/translations/ko-KR/content/github/developing-online-with-codespaces/using-codespaces-in-visual-studio.md
@@ -46,8 +46,12 @@ Connecting to {% data variables.product.prodname_github_codespaces %} with the
### Configuring a codespace for {% data variables.product.prodname_vs %}
-The default codespace environment created by {% data variables.product.prodname_vs %} includes popular frameworks and tools such as .NET Core, Microsoft SQL Server, Python, and the Windows SDK. {% data variables.product.prodname_github_codespaces %} created with {% data variables.product.prodname_vs %} can be customized through a subset of `devcontainers.json` properties and a new tool called devinit, included with {% data variables.product.prodname_vs %}.
+A codespace, created with {% data variables.product.prodname_vs %}, can be customized through a new tool called devinit, a command line tool included with {% data variables.product.prodname_vs %}.
#### devinit
-The [devinit](https://docs.microsoft.com/visualstudio/devinit/getting-started-with-devinit) command-line tool lets you install additional frameworks and tools into your Windows development codespaces, as well as run PowerShell scripts or modify environment variables. devinit supports a configuration file called [devinit.json](https://docs.microsoft.com/visualstudio/devinit/devinit-json), which can be added to your project for creating customized and repeatable development environments. For more information about Windows codespace configuration and devinit, see [Customize a codespace](https://docs.microsoft.com/visualstudio/ide/codespaces/customize-codespaces) in the {% data variables.product.prodname_vs %} documentation.
+[devinit](https://docs.microsoft.com/visualstudio/devinit/getting-started-with-devinit) lets you install additional frameworks and tools into your Windows development codespaces, modify environment variables, and more.
+
+devinit supports a configuration file called [devinit.json](https://docs.microsoft.com/visualstudio/devinit/devinit-json). You can add this file to your project if you want to create a customized and repeatable development environment. When you use devinit with a [devcontainer.json](https://docs.microsoft.com/visualstudio/ide/codespaces/customize-codespaces#running-devinit-when-creating-a-codespace) file, your codespaces will be automatically configured on creation.
+
+For more information about Windows codespace configuration and devinit, see [Customize a codespace](https://docs.microsoft.com/visualstudio/ide/codespaces/customize-codespaces) in the {% data variables.product.prodname_vs %} documentation. For more information about devinit, see [Getting started with devinit](https://docs.microsoft.com/visualstudio/devinit/getting-started-with-devinit).
diff --git a/translations/ko-KR/content/github/getting-started-with-github/create-a-repo.md b/translations/ko-KR/content/github/getting-started-with-github/create-a-repo.md
index 4d0dd3551a1d..20e8bd22c874 100644
--- a/translations/ko-KR/content/github/getting-started-with-github/create-a-repo.md
+++ b/translations/ko-KR/content/github/getting-started-with-github/create-a-repo.md
@@ -10,14 +10,26 @@ versions:
github-ae: '*'
---
+{% if currentVersion == "free-pro-team@latest" %}
+
You can store a variety of projects in {% data variables.product.product_name %} repositories, including open source projects. With [open source projects](http://opensource.org/about), you can share code to make better, more reliable software.
+{% elsif enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
+
+You can store a variety of projects in {% data variables.product.product_name %} repositories, including innersource projects. With innersource, you can share code to make better, more reliable software. For more information on innersource, see {% data variables.product.company_short %}'s whitepaper "[An introduction to innersource](https://resources.github.com/whitepapers/introduction-to-innersource/)."
+
+{% endif %}
+
+{% if currentVersion == "free-pro-team@latest" %}
+
{% note %}
**Note:** You can create public repositories for an open source project. When creating your public repository, make sure to include a [license file](http://choosealicense.com/) that determines how you want your project to be shared with others. {% data reusables.open-source.open-source-guide-repositories %} {% data reusables.open-source.open-source-learning-lab %}
{% endnote %}
+{% endif %}
+
{% data reusables.repositories.create_new %}
2. Type a short, memorable name for your repository. For example, "hello-world". 
3. Optionally, add a description of your repository. For example, "My first repository on
diff --git a/translations/ko-KR/content/github/getting-started-with-github/fork-a-repo.md b/translations/ko-KR/content/github/getting-started-with-github/fork-a-repo.md
index 0b21acee3169..932c7c56c92b 100644
--- a/translations/ko-KR/content/github/getting-started-with-github/fork-a-repo.md
+++ b/translations/ko-KR/content/github/getting-started-with-github/fork-a-repo.md
@@ -25,10 +25,16 @@ For example, you can use forks to propose changes related to fixing a bug. Rathe
Open source software is based on the idea that by sharing code, we can make better, more reliable software. For more information, see the "[About the Open Source Initiative](http://opensource.org/about)" on the Open Source Initiative.
+For more information about applying open source principles to your organization's development work on {% data variables.product.product_location %}, see {% data variables.product.prodname_dotcom %}'s whitepaper "[An introduction to innersource](https://resources.github.com/whitepapers/introduction-to-innersource/)."
+
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
When creating your public repository from a fork of someone's project, make sure to include a license file that determines how you want your project to be shared with others. For more information, see "[Choose an open source license](http://choosealicense.com/)" at choosealicense.
{% data reusables.open-source.open-source-guide-repositories %} {% data reusables.open-source.open-source-learning-lab %}
+{% endif %}
+
{% note %}
**Note**: {% data reusables.repositories.desktop-fork %}
diff --git a/translations/ko-KR/content/github/getting-started-with-github/setting-up-a-trial-of-github-enterprise-server.md b/translations/ko-KR/content/github/getting-started-with-github/setting-up-a-trial-of-github-enterprise-server.md
index 259e8566da9a..06f35c6440b6 100644
--- a/translations/ko-KR/content/github/getting-started-with-github/setting-up-a-trial-of-github-enterprise-server.md
+++ b/translations/ko-KR/content/github/getting-started-with-github/setting-up-a-trial-of-github-enterprise-server.md
@@ -36,7 +36,7 @@ To get the most out of your trial, follow these steps:
- [Quick start guide to {% data variables.product.prodname_dotcom %}](https://resources.github.com/webcasts/Quick-start-guide-to-GitHub/) webcast
- [Understanding the {% data variables.product.prodname_dotcom %} flow](https://guides.github.com/introduction/flow/) in {% data variables.product.prodname_dotcom %} Guides
- [Hello World](https://guides.github.com/activities/hello-world/) in {% data variables.product.prodname_dotcom %} Guides
-3. To configure your instance to meet your organization's needs, see "[Configuring your enterprise](/admin/configuration/configuring-your-enterprise)."
+3. To configure your instance to meet your organization's needs, see "[Configuring your enterprise](/enterprise/admin/configuration/configuring-your-enterprise)."
4. To integrate {% data variables.product.prodname_ghe_server %} with your identity provider, see "[Using SAML](/enterprise/admin/user-management/using-saml)" and "[Using LDAP](/enterprise/admin/authentication/using-ldap)."
5. Invite an unlimited number of people to join your trial.
- Add users to your {% data variables.product.prodname_ghe_server %} instance using built-in authentication or your configured identity provider. For more information, see "[Using built in authentication](/enterprise/admin/user-management/using-built-in-authentication)."
diff --git a/translations/ko-KR/content/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies.md b/translations/ko-KR/content/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies.md
index 6f58c5039d4f..a17fe4c6a178 100644
--- a/translations/ko-KR/content/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies.md
+++ b/translations/ko-KR/content/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies.md
@@ -75,7 +75,7 @@ You can see all of the alerts that affect a particular project{% if currentVersi
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
By default, we notify people with admin permissions in the affected repositories about new
-{% data variables.product.prodname_dependabot_alerts %}.{% endif %} {% if currentVersion == "free-pro-team@latest" %}{% data variables.product.product_name %} never publicly discloses identified vulnerabilities for any repository. You can also make {% data variables.product.prodname_dependabot_alerts %} visible to additional people or teams working repositories that you own or have admin permissions for. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-dependabot-alerts)."
+{% data variables.product.prodname_dependabot_alerts %}.{% endif %} {% if currentVersion == "free-pro-team@latest" %}{% data variables.product.product_name %} never publicly discloses identified vulnerabilities for any repository. You can also make {% data variables.product.prodname_dependabot_alerts %} visible to additional people or teams working repositories that you own or have admin permissions for. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-security-alerts)."
{% endif %}
{% if enterpriseServerVersions contains currentVersion and currentVersion ver_lt "enterprise-server@2.22" %}
diff --git a/translations/ko-KR/content/github/managing-subscriptions-and-notifications-on-github/about-notifications.md b/translations/ko-KR/content/github/managing-subscriptions-and-notifications-on-github/about-notifications.md
index 337a63de5e0d..3c15a48c5431 100644
--- a/translations/ko-KR/content/github/managing-subscriptions-and-notifications-on-github/about-notifications.md
+++ b/translations/ko-KR/content/github/managing-subscriptions-and-notifications-on-github/about-notifications.md
@@ -21,7 +21,12 @@ You can choose to subscribe to notifications for:
- A conversation in a specific issue, pull request, or gist.
- All activity in a repository or team discussion.
- CI activity, such as the status of workflows in repositories set up with {% data variables.product.prodname_actions %}.
+{% if currentVersion == "free-pro-team@latest" %}
+- Issues, pulls requests, releases and discussions (if enabled) in a repository.
+{% endif %}
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}
- Releases in a repository.
+{% endif %}
You can also choose to automatically watch all repositories that you have push access to, except forks. You can watch any other repository you have access to manually by clicking **Watch**.
diff --git a/translations/ko-KR/content/github/managing-subscriptions-and-notifications-on-github/configuring-notifications.md b/translations/ko-KR/content/github/managing-subscriptions-and-notifications-on-github/configuring-notifications.md
index 4a9b49d8a6d6..b229070b4fd0 100644
--- a/translations/ko-KR/content/github/managing-subscriptions-and-notifications-on-github/configuring-notifications.md
+++ b/translations/ko-KR/content/github/managing-subscriptions-and-notifications-on-github/configuring-notifications.md
@@ -21,16 +21,17 @@ versions:
### Notification delivery options
-You have three basic options for notification delivery:
- - the notifications inbox on {% data variables.product.product_name %}{% if currentVersion == "free-pro-team@latest" %}
- - the notifications inbox on {% data variables.product.prodname_mobile %}, which syncs with the inbox on {% data variables.product.product_name %}{% endif %}
- - an email client that uses a verified email address, which can also sync with the notifications inbox on {% data variables.product.product_name %}{% if currentVersion == "free-pro-team@latest" %} and {% data variables.product.prodname_mobile %}{% endif %}
+You can receive notifications for activity on {% data variables.product.product_name %} in the following locations.
+
+ - The notifications inbox in the {% data variables.product.product_name %} web interface{% if currentVersion == "free-pro-team@latest" %}
+ - The notifications inbox on {% data variables.product.prodname_mobile %}, which syncs with the inbox on {% data variables.product.product_name %}{% endif %}
+ - An email client that uses a verified email address, which can also sync with the notifications inbox on {% data variables.product.product_name %}{% if currentVersion == "free-pro-team@latest" %} and {% data variables.product.prodname_mobile %}{% endif %}
{% if currentVersion == "free-pro-team@latest" %}
{% data reusables.notifications-v2.notifications-inbox-required-setting %} For more information, see "[Choosing your notification settings](#choosing-your-notification-settings)."
{% endif %}
-{% data reusables.notifications-v2.tip-for-syncing-email-and-your-inbox-on-github %}
+{% data reusables.notifications.shared_state %}
#### Benefits of the notifications inbox
@@ -59,8 +60,21 @@ Email notifications also allow flexibility with the types of notifications you r
### About participating and watching notifications
-When you watch a repository, you're subscribing to updates for activity in that repository. Similarly, when you watch a specific team's discussions, you're subscribing to all conversation updates on that team's page. To see repositories that you're watching, see [https://github.com/watching](https://github.com/watching). For more information, see "[Managing subscriptions and notifications on GitHub](/github/managing-subscriptions-and-notifications-on-github/managing-subscriptions-for-activity-on-github)."
+When you watch a repository, you're subscribing to updates for activity in that repository. Similarly, when you watch a specific team's discussions, you're subscribing to all conversation updates on that team's page. For more information, see "[About team discussions](/github/building-a-strong-community/about-team-discussions)."
+
+To see repositories that you're watching, go to your [watching page](https://github.com/watching). For more information, see "[Managing subscriptions and notifications on GitHub](/github/managing-subscriptions-and-notifications-on-github/managing-subscriptions-for-activity-on-github)."
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}
+#### Configuring notifications
+{% endif %}
+You can configure notifications for a repository on the repository page, or on your watching page.
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %} You can choose to only receive notifications for releases in a repository, or ignore all notifications for a repository.{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+
+#### About custom notifications
+{% data reusables.notifications-v2.custom-notifications-beta %}
+You can customize notifications for a repository, for example, you can choose to only be notified when updates to one or more types of events (issues, pull request, releases, discussions) happen within a repository, or ignore all notifications for a repository.
+{% endif %} For more information, see "[Viewing your subscriptions](/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions#configuring-your-watch-settings-for-an-individual-repository)."
+#### Participating in conversations
Anytime you comment in a conversation or when someone @mentions your username, you are _participating_ in a conversation. By default, you are automatically subscribed to a conversation when you participate in it. You can unsubscribe from a conversation you've participated in manually by clicking **Unsubscribe** on the issue or pull request or through the **Unsubscribe** option in the notifications inbox.
For conversations you're watching or participating in, you can choose whether you want to receive notifications by email or through the notifications inbox on {% data variables.product.product_name %}{% if currentVersion == "free-pro-team@latest" %} and {% data variables.product.prodname_mobile %}{% endif %}.
@@ -95,7 +109,9 @@ Choose a default email address where you want to send updates for conversations
- Pull request pushes.
- Your own updates, such as when you open, comment on, or close an issue or pull request.
-Depending on the organization that owns the repository, you can also send notifications to different email addresses for specific repositories. For example, you can send notifications for a specific public repository to a verified personal email address. Your organization may require the email address to be verified for a specific domain. For more information, see “[Choosing where your organization’s email notifications are sent](/github/managing-subscriptions-and-notifications-on-github/configuring-notifications#choosing-where-your-organizations-email-notifications-are-sent)."
+Depending on the organization that owns the repository, you can also send notifications to different email addresses. Your organization may require the email address to be verified for a specific domain. For more information, see "[Choosing where your organization’s email notifications are sent](/github/managing-subscriptions-and-notifications-on-github/configuring-notifications#choosing-where-your-organizations-email-notifications-are-sent)."
+
+You can also send notifications for a specific repository to an email address. For more information, see "[About email notifications for pushes to your repository](/github/administering-a-repository/about-email-notifications-for-pushes-to-your-repository)."
{% data reusables.notifications-v2.email-notification-caveats %}
diff --git a/translations/ko-KR/content/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions.md b/translations/ko-KR/content/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions.md
index b038653bde09..4cb5408b6384 100644
--- a/translations/ko-KR/content/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions.md
+++ b/translations/ko-KR/content/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions.md
@@ -55,6 +55,13 @@ When you unwatch a repository, you unsubscribe from future updates from that rep
{% data reusables.notifications.access_notifications %}
1. In the left sidebar, under the list of repositories, use the "Manage notifications" drop-down to click **Watched repositories**. 
2. On the watched repositories page, after you've evaluated the repositories you're watching, choose whether to:
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}
- unwatch a repository
- only watch releases for a repository
- ignore all notifications for a repository
+{% endif %}
+{% if currentVersion == "free-pro-team@latest" %}
+ - unwatch a repository
+ - ignore all notifications for a repository
+ - customize the types of event you receive notifications for (issues, pull requests, releases or discussions, if enabled)
+{% endif %}
\ No newline at end of file
diff --git a/translations/ko-KR/content/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions.md b/translations/ko-KR/content/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions.md
index 1daafdceacd0..85c5c093e6c6 100644
--- a/translations/ko-KR/content/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions.md
+++ b/translations/ko-KR/content/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions.md
@@ -32,7 +32,16 @@ When your inbox has too many notifications to manage, consider whether you have
For more information, see "[Configuring notifications](/github/managing-subscriptions-and-notifications-on-github/configuring-notifications#automatic-watching)."
-To see an overview of your repository subscriptions, see "[Reviewing repositories that you're watching](#reviewing-repositories-that-youre-watching)." Many people forget about repositories that they've chosen to watch in the past. From the "Watched repositories" page you can quickly unwatch repositories. For more information on ways to unsubscribe, see "[Managing subscriptions](/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions)."
+To see an overview of your repository subscriptions, see "[Reviewing repositories that you're watching](#reviewing-repositories-that-youre-watching)."
+{% if currentVersion == "free-pro-team@latest" %}
+{% tip %}
+
+**Tip:** You can select the types of event to be notified of by using the **Custom** option of the **Watch/Unwatch** dropdown list in your [watching page](https://github.com/watching) or on any repository page on {% data variables.product.prodname_dotcom_the_website %}. For more information, see "[Configuring your watch settings for an individual repository](#configuring-your-watch-settings-for-an-individual-repository)" below.
+
+{% endtip %}
+{% endif %}
+
+Many people forget about repositories that they've chosen to watch in the past. From the "Watched repositories" page you can quickly unwatch repositories. For more information on ways to unsubscribe, see "[Unwatch recommendations](https://github.blog/changelog/2020-11-10-unwatch-recommendations/)" on {% data variables.product.prodname_blog %} and "[Managing your subscriptions](/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions)." You can also create a triage worflow to help with the notifications you receive. For guidance on triage workflows, see "[Customizing a workflow for triaging your notifications](/github/managing-subscriptions-and-notifications-on-github/customizing-a-workflow-for-triaging-your-notifications)."
### Reviewing all of your subscriptions
@@ -55,20 +64,38 @@ To see an overview of your repository subscriptions, see "[Reviewing repositorie
### Reviewing repositories that you're watching
1. In the left sidebar, under the list of repositories, use the "Manage notifications" drop-down menu and click **Watched repositories**. 
-
-3. Evaluate the repositories that you are watching and decide if their updates are still relevant and helpful. When you watch a repository, you will be notified of all conversations for that repository.
-
+2. Evaluate the repositories that you are watching and decide if their updates are still relevant and helpful. When you watch a repository, you will be notified of all conversations for that repository.
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}

+{% endif %}
+{% if currentVersion == "free-pro-team@latest" %}
+ 
+{% endif %}
{% tip %}
- **Tip:** Instead of watching a repository, consider only watching releases for a repository or completely unwatching a repository. When you unwatch a repository, you can still be notified when you're @mentioned or participating in a thread. When you only watch releases for a repository, you're only notified when there's a new release, you're participating in a thread, or you or a team you're on is @mentioned.
+ **Tip:** Instead of watching a repository, consider only receiving notifications {% if currentVersion == "free-pro-team@latest" %}when there are updates to issues, pull requests, releases or discussions (if enabled for the repository), or any combination of these options,{% else %}for releases in a repository,{% endif %} or completely unwatching a repository.
+
+ When you unwatch a repository, you can still be notified when you're @mentioned or participating in a thread. When you configure to receive notifications for certain event types, you're only notified when there are updates to these event types in the repository, you're participating in a thread, or you or a team you're on is @mentioned.
{% endtip %}
### Configuring your watch settings for an individual repository
-You can choose whether to watch or unwatch an individual repository. You can also choose to only be notified of new releases or ignore an individual repository.
+You can choose whether to watch or unwatch an individual repository. You can also choose to only be notified of {% if currentVersion == "free-pro-team@latest" %}certain event types such as issues, pull requests, discussions (if enabled for the repository) and {% endif %}new releases, or completely ignore an individual repository.
{% data reusables.repositories.navigate-to-repo %}
-2. In the upper-right corner, click the "Watch" drop-down menu to select a watch option. 
+2. In the upper-right corner, click the "Watch" drop-down menu to select a watch option.
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}
+ 
+{% endif %}
+{% if currentVersion == "free-pro-team@latest" %}
+ 
+{% data reusables.notifications-v2.custom-notifications-beta %}
+The **Custom** option allows you to further customize notifications so that you're only notified when specific events happen in the repository, in addition to participating and @mentions.
+
+ 
+
+If you select "Issues", you will be notified about, and subscribed to, updates on every issue (including those that existed prior to you selecting this option) in the repository. If you're @mentioned in a pull request in this repository, you'll receive notifications for that too, and you'll be subscribed to updates on that specific pull request, in addition to being notified about issues.
+
+{% endif %}
diff --git a/translations/ko-KR/content/github/managing-your-work-on-github/creating-an-issue.md b/translations/ko-KR/content/github/managing-your-work-on-github/creating-an-issue.md
index 153fdfaddb0a..83477137fb11 100644
--- a/translations/ko-KR/content/github/managing-your-work-on-github/creating-an-issue.md
+++ b/translations/ko-KR/content/github/managing-your-work-on-github/creating-an-issue.md
@@ -1,6 +1,7 @@
---
title: Creating an issue
intro: 'Issues can be used to keep track of bugs, enhancements, or other requests.'
+permissions: 'People with read permissions can create an issue in a repository where issues are enabled.'
redirect_from:
- /articles/creating-an-issue
versions:
@@ -9,8 +10,6 @@ versions:
github-ae: '*'
---
-{% data reusables.repositories.create-issue-in-public-repository %}
-
You can open a new issue based on code from an existing pull request. For more information, see "[Opening an issue from code](/github/managing-your-work-on-github/opening-an-issue-from-code)."
You can open a new issue directly from a comment in an issue or a pull request review. For more information, see "[Opening an issue from a comment](/github/managing-your-work-on-github/opening-an-issue-from-a-comment)."
diff --git a/translations/ko-KR/content/github/managing-your-work-on-github/opening-an-issue-from-a-comment.md b/translations/ko-KR/content/github/managing-your-work-on-github/opening-an-issue-from-a-comment.md
index 62e9dd1f1b94..d7615598af2a 100644
--- a/translations/ko-KR/content/github/managing-your-work-on-github/opening-an-issue-from-a-comment.md
+++ b/translations/ko-KR/content/github/managing-your-work-on-github/opening-an-issue-from-a-comment.md
@@ -1,6 +1,7 @@
---
title: Opening an issue from a comment
intro: You can open a new issue from a specific comment in an issue or pull request.
+permissions: 'People with read permissions can create an issue in a repository where issues are enabled.'
versions:
free-pro-team: '*'
enterprise-server: '*'
@@ -9,6 +10,8 @@ versions:
When you open an issue from a comment, the issue contains a snippet showing where the comment was originally posted.
+{% data reusables.repositories.administrators-can-disable-issues %}
+
1. Navigate to the comment you'd like to open an issue from.
2. In that comment, click {% octicon "kebab-horizontal" aria-label="The horizontal kebab icon" %}. 
diff --git a/translations/ko-KR/content/github/managing-your-work-on-github/opening-an-issue-from-code.md b/translations/ko-KR/content/github/managing-your-work-on-github/opening-an-issue-from-code.md
index 81273e336a76..539dc82b4966 100644
--- a/translations/ko-KR/content/github/managing-your-work-on-github/opening-an-issue-from-code.md
+++ b/translations/ko-KR/content/github/managing-your-work-on-github/opening-an-issue-from-code.md
@@ -1,6 +1,7 @@
---
title: Opening an issue from code
intro: You can open a new issue from a specific line or lines of code in a file or pull request.
+permissions: 'People with read permissions can create an issue in a repository where issues are enabled.'
redirect_from:
- /articles/opening-an-issue-from-code
versions:
@@ -13,7 +14,7 @@ When you open an issue from code, the issue contains a snippet showing the line

-{% data reusables.repositories.create-issue-in-public-repository %}
+{% data reusables.repositories.administrators-can-disable-issues %}
{% data reusables.repositories.navigate-to-repo %}
2. Locate the code you want to reference in an issue:
diff --git a/translations/ko-KR/content/github/managing-your-work-on-github/transferring-an-issue-to-another-repository.md b/translations/ko-KR/content/github/managing-your-work-on-github/transferring-an-issue-to-another-repository.md
index 475d6aa20156..089cd7a04e13 100644
--- a/translations/ko-KR/content/github/managing-your-work-on-github/transferring-an-issue-to-another-repository.md
+++ b/translations/ko-KR/content/github/managing-your-work-on-github/transferring-an-issue-to-another-repository.md
@@ -11,7 +11,7 @@ versions:
To transfer an open issue to another repository, you must have write permissions on the repository the issue is in and the repository you're transferring the issue to. For more information, see "[Repository permission levels for an organization](/articles/repository-permission-levels-for-an-organization)."
-You can only transfer issues between repositories owned by the same user or organization account. You can't transfer an issue from a private repository to a public repository.
+You can only transfer issues between repositories owned by the same user or organization account.{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}You can't transfer an issue from a private repository to a public repository.{% endif %}
When you transfer an issue, comments and assignees are retained. The issue's labels and milestones are not retained. This issue will stay on any user-owned or organization-wide project boards and be removed from any repository project boards. For more information, see "[About project boards](/articles/about-project-boards)."
diff --git a/translations/ko-KR/content/github/searching-for-information-on-github/about-searching-on-github.md b/translations/ko-KR/content/github/searching-for-information-on-github/about-searching-on-github.md
index c880c29e2f67..2f84601561ad 100644
--- a/translations/ko-KR/content/github/searching-for-information-on-github/about-searching-on-github.md
+++ b/translations/ko-KR/content/github/searching-for-information-on-github/about-searching-on-github.md
@@ -14,7 +14,7 @@ versions:
github-ae: '*'
---
-You can search globally across all of {% data variables.product.product_name %}, or scope your search to a particular repository or organization.
+{% data reusables.search.you-can-search-globally %}
- To search globally across all of {% data variables.product.product_name %}, type what you're looking for into the search field at the top of any page, and choose "All {% data variables.product.prodname_dotcom %}" in the search drop-down menu.
- To search within a particular repository or organization, navigate to the repository or organization page, type what you're looking for into the search field at the top of the page, and press **Enter**.
@@ -23,7 +23,8 @@ You can search globally across all of {% data variables.product.product_name %},
**참고:**
-- {% data reusables.search.required_login %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- {% data reusables.search.required_login %}{% endif %}
- {% data variables.product.prodname_pages %} sites are not searchable on {% data variables.product.product_name %}. However you can search the source content if it exists in the default branch of a repository, using code search. For more information, see "[Searching code](/articles/searching-code)." For more information about {% data variables.product.prodname_pages %}, see "[What is GitHub Pages?](/articles/what-is-github-pages/)"
- Currently our search doesn't support exact matching.
- Whenever you are searching in code files, only the first two results in each file will be returned.
@@ -36,7 +37,7 @@ After running a search on {% data variables.product.product_name %}, you can sor
### Types of searches on {% data variables.product.prodname_dotcom %}
-You can search the following types of information across all public {% data variables.product.product_name %} repositories, and all private {% data variables.product.product_name %} repositories you have access to:
+You can search for the following information across all repositories you can access on {% data variables.product.product_location %}.
- [Repositories](/articles/searching-for-repositories)
- [Topics](/articles/searching-topics)
diff --git a/translations/ko-KR/content/github/searching-for-information-on-github/searching-code.md b/translations/ko-KR/content/github/searching-for-information-on-github/searching-code.md
index be4c5ca57e82..62ea1db6cef8 100644
--- a/translations/ko-KR/content/github/searching-for-information-on-github/searching-code.md
+++ b/translations/ko-KR/content/github/searching-for-information-on-github/searching-code.md
@@ -11,7 +11,7 @@ versions:
github-ae: '*'
---
-You can search for code globally across all of {% data variables.product.product_name %}, or search for code within a particular repository or organization. To search for code across all public repositories, you must be signed in to a {% data variables.product.product_name %} account. For more information, see "[About searching on GitHub](/articles/about-searching-on-github)."
+{% data reusables.search.you-can-search-globally %} For more information, see "[About searching on GitHub](/articles/about-searching-on-github)."
You can only search code using these code search qualifiers. Search qualifiers specifically for repositories, users, or commits, will not work when searching for code.
@@ -21,13 +21,13 @@ You can only search code using these code search qualifiers. Search qualifiers s
Due to the complexity of searching code, there are some restrictions on how searches are performed:
-- {% data reusables.search.required_login %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- {% data reusables.search.required_login %}{% endif %}
- Code in [forks](/articles/about-forks) is only searchable if the fork has more stars than the parent repository. Forks with fewer stars than the parent repository are **not** indexed for code search. To include forks with more stars than their parent in the search results, you will need to add `fork:true` or `fork:only` to your query. For more information, see "[Searching in forks](/articles/searching-in-forks)."
- Only the _default branch_ is indexed for code search.{% if currentVersion == "free-pro-team@latest" %}
- Only files smaller than 384 KB are searchable.{% else %}* Only files smaller than 5 MB are searchable.
- Only the first 500 KB of each file is searchable.{% endif %}
- Only repositories with fewer than 500,000 files are searchable.
-- Users who are signed in can search all public repositories.
- Except with [`filename`](#search-by-filename) searches, you must always include at least one search term when searching source code. For example, searching for [`language:javascript`](https://github.com/search?utf8=%E2%9C%93&q=language%3Ajavascript&type=Code&ref=searchresults) is not valid, while [`amazing language:javascript`](https://github.com/search?utf8=%E2%9C%93&q=amazing+language%3Ajavascript&type=Code&ref=searchresults) is.
- At most, search results can show two fragments from the same file, but there may be more results within the file.
- You can't use the following wildcard characters as part of your search query: . , : ; / \ ` ' " = * ! ? # $ & + ^ | ~ < > ( ) { } [ ]
. The search will simply ignore these symbols.
diff --git a/translations/ko-KR/content/github/searching-for-information-on-github/searching-commits.md b/translations/ko-KR/content/github/searching-for-information-on-github/searching-commits.md
index 692aa3ec0da9..3c370b8deed3 100644
--- a/translations/ko-KR/content/github/searching-for-information-on-github/searching-commits.md
+++ b/translations/ko-KR/content/github/searching-for-information-on-github/searching-commits.md
@@ -96,14 +96,11 @@ To search commits in all repositories owned by a certain user or organization, u
| org:ORGNAME
| [**test org:github**](https://github.com/search?utf8=%E2%9C%93&q=test+org%3Agithub&type=Commits) matches commit messages with the word "test" in repositories owned by @github. |
| repo:USERNAME/REPO
| [**language repo:defunkt/gibberish**](https://github.com/search?utf8=%E2%9C%93&q=language+repo%3Adefunkt%2Fgibberish&type=Commits) matches commit messages with the word "language" in @defunkt's "gibberish" repository. |
-### Filter public or private repositories
+### Filter by repository visibility
-The `is` qualifier matches public or private commits.
+The `is` qualifier matches commits from repositories with the specified visibility. For more information, see "[About repository visibility](/github/creating-cloning-and-archiving-repositories/about-repository-visibility).
-| Qualifier | 예시 |
-| ------------ | ------------------------------------------------------------------------------------------------ |
-| `is:public` | [**is:public**](https://github.com/search?q=is%3Apublic&type=Commits) matches public commits. |
-| `is:private` | [**is:private**](https://github.com/search?q=is%3Aprivate&type=Commits) matches private commits. |
+| Qualifier | Example | ------------- | ------------- |{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %} | `is:public` | [**is:public**](https://github.com/search?q=is%3Apublic&type=Commits) matches commits to public repositories.{% endif %} | `is:internal` | [**is:internal**](https://github.com/search?q=is%3Ainternal&type=Commits) matches commits to internal repositories. | `is:private` | [**is:private**](https://github.com/search?q=is%3Aprivate&type=Commits) matches commits to private repositories.
### 더 읽을거리
diff --git a/translations/ko-KR/content/github/searching-for-information-on-github/searching-for-repositories.md b/translations/ko-KR/content/github/searching-for-information-on-github/searching-for-repositories.md
index 3b7b8df50920..b71ed8864c3c 100644
--- a/translations/ko-KR/content/github/searching-for-information-on-github/searching-for-repositories.md
+++ b/translations/ko-KR/content/github/searching-for-information-on-github/searching-for-repositories.md
@@ -10,7 +10,7 @@ versions:
github-ae: '*'
---
-You can search for repositories globally across all of {% data variables.product.product_name %}, or search for repositories within a particular organization. For more information, see "[About searching on {% data variables.product.prodname_dotcom %}](/articles/about-searching-on-github)."
+You can search for repositories globally across all of {% data variables.product.product_location %}, or search for repositories within a particular organization. For more information, see "[About searching on {% data variables.product.prodname_dotcom %}](/articles/about-searching-on-github)."
To include forks in the search results, you will need to add `fork:true` or `fork:only` to your query. For more information, see "[Searching in forks](/articles/searching-in-forks)."
@@ -20,22 +20,22 @@ To include forks in the search results, you will need to add `fork:true` or `for
With the `in` qualifier you can restrict your search to the repository name, repository description, contents of the README file, or any combination of these. When you omit this qualifier, only the repository name and description are searched.
-| Qualifier | 예시 |
-| ----------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `in:name` | [**jquery in:name**](https://github.com/search?q=jquery+in%3Aname&type=Repositories) matches repositories with "jquery" in their name. |
-| `in:description` | [**jquery in:name,description**](https://github.com/search?q=jquery+in%3Aname%2Cdescription&type=Repositories) matches repositories with "jquery" in their name or description. |
-| `in:readme` | [**jquery in:readme**](https://github.com/search?q=jquery+in%3Areadme&type=Repositories) matches repositories mentioning "jquery" in their README file. |
-| `repo:owner/name` | [**repo:octocat/hello-world**](https://github.com/search?q=repo%3Aoctocat%2Fhello-world) matches a specific repository name. |
+| Qualifier | 예시 |
+| ----------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `in:name` | [**jquery in:name**](https://github.com/search?q=jquery+in%3Aname&type=Repositories) matches repositories with "jquery" in the repository name. |
+| `in:description` | [**jquery in:name,description**](https://github.com/search?q=jquery+in%3Aname%2Cdescription&type=Repositories) matches repositories with "jquery" in the repository name or description. |
+| `in:readme` | [**jquery in:readme**](https://github.com/search?q=jquery+in%3Areadme&type=Repositories) matches repositories mentioning "jquery" in the repository's README file. |
+| `repo:owner/name` | [**repo:octocat/hello-world**](https://github.com/search?q=repo%3Aoctocat%2Fhello-world) matches a specific repository name. |
### Search based on the contents of a repository
-You can find a repository by searching for content in its README file, using the `in:readme` qualifier.
+You can find a repository by searching for content in the repository's README file using the `in:readme` qualifier. For more information, see "[About READMEs](/github/creating-cloning-and-archiving-repositories/about-readmes)."
Besides using `in:readme`, it's not possible to find repositories by searching for specific content within the repository. To search for a specific file or content within a repository, you can use the file finder or code-specific search qualifiers. For more information, see "[Finding files on {% data variables.product.prodname_dotcom %}](/articles/finding-files-on-github)" and "[Searching code](/articles/searching-code)."
-| Qualifier | 예시 |
-| ----------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `in:readme` | [**octocat in:readme**](https://github.com/search?q=octocat+in%3Areadme&type=Repositories) matches repositories mentioning "octocat" in their README file. |
+| Qualifier | 예시 |
+| ----------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `in:readme` | [**octocat in:readme**](https://github.com/search?q=octocat+in%3Areadme&type=Repositories) matches repositories mentioning "octocat" in the repository's README file. |
### Search within a user's or organization's repositories
@@ -48,7 +48,7 @@ To search in all repositories owned by a certain user or organization, you can u
### Search by repository size
-The `size` qualifier finds repositories that match a certain size (in kilobytes), using [greater than, less than, and range qualifiers](/articles/understanding-the-search-syntax).
+The `size` qualifier finds repositories that match a certain size (in kilobytes), using greater than, less than, and range qualifiers. For more information, see "[Understanding the search syntax](/github/searching-for-information-on-github/understanding-the-search-syntax)."
| Qualifier | 예시 |
| ------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -59,7 +59,7 @@ The `size` qualifier finds repositories that match a certain size (in kilobytes)
### Search by number of followers
-You can filter repositories based on the number of followers that they have, using the `followers` qualifier with [greater than, less than, and range qualifiers](/articles/understanding-the-search-syntax).
+You can filter repositories based on the number of users who follow the repositories, using the `followers` qualifier with greater than, less than, and range qualifiers. For more information, see "[Understanding the search syntax](/github/searching-for-information-on-github/understanding-the-search-syntax)."
| Qualifier | 예시 |
| ------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -68,7 +68,7 @@ You can filter repositories based on the number of followers that they have, usi
### Search by number of forks
-The `forks` qualifier specifies the number of forks a repository should have, using [greater than, less than, and range qualifiers](/articles/understanding-the-search-syntax).
+The `forks` qualifier specifies the number of forks a repository should have, using greater than, less than, and range qualifiers. For more information, see "[Understanding the search syntax](/github/searching-for-information-on-github/understanding-the-search-syntax)."
| Qualifier | 예시 |
| ------------------------- | ----------------------------------------------------------------------------------------------------------------------------------------- |
@@ -79,7 +79,7 @@ The `forks` qualifier specifies the number of forks a repository should have, us
### Search by number of stars
-You can search repositories based on the number of [stars](/articles/saving-repositories-with-stars) a repository has, using [greater than, less than, and range qualifiers](/articles/understanding-the-search-syntax)
+You can search repositories based on the number of stars the repositories have, using greater than, less than, and range qualifiers. For more information, see "[Saving repositories with stars](/github/getting-started-with-github/saving-repositories-with-stars)" and "[Understanding the search syntax](/github/searching-for-information-on-github/understanding-the-search-syntax)."
| Qualifier | 예시 |
| ------------------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -103,7 +103,7 @@ Both take a date as a parameter. {% data reusables.time_date.date_format %} {% d
### Search by language
-You can search repositories based on the main language they're written in.
+You can search repositories based on the language of the code in the repositories.
| Qualifier | 예시 |
| ------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -111,7 +111,7 @@ You can search repositories based on the main language they're written in.
### Search by topic
-You can find all of the repositories that are classified with a particular [topic](/articles/classifying-your-repository-with-topics).
+You can find all of the repositories that are classified with a particular topic. For more information, see "[Classifying your repository with topics](/github/administering-a-repository/classifying-your-repository-with-topics)."
| Qualifier | 예시 |
| ------------------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -119,35 +119,36 @@ You can find all of the repositories that are classified with a particular [topi
### Search by number of topics
-You can search repositories by the number of [topics](/articles/classifying-your-repository-with-topics) that have been applied to them, using the `topics` qualifier along with [greater than, less than, and range qualifiers](/articles/understanding-the-search-syntax).
+You can search repositories by the number of topics that have been applied to the repositories, using the `topics` qualifier along with greater than, less than, and range qualifiers. For more information, see "[Classifying your repository with topics](/github/administering-a-repository/classifying-your-repository-with-topics)" and "[Understanding the search syntax](/github/searching-for-information-on-github/understanding-the-search-syntax)."
| Qualifier | 예시 |
| -------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| topics:n
| [**topics:5**](https://github.com/search?utf8=%E2%9C%93&q=topics%3A5&type=Repositories&ref=searchresults) matches repositories that have five topics. |
| | [**topics:>3**](https://github.com/search?utf8=%E2%9C%93&q=topics%3A%3E3&type=Repositories&ref=searchresults) matches repositories that have more than three topics. |
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
### Search by license
-You can search repositories by their [license](/articles/licensing-a-repository). You must use a [license keyword](/articles/licensing-a-repository/#searching-github-by-license-type) to filter repositories by a particular license or license family.
+You can search repositories by the type of license in the repositories. You must use a license keyword to filter repositories by a particular license or license family. For more information, see "[Licensing a repository](/github/creating-cloning-and-archiving-repositories/licensing-a-repository)."
| Qualifier | 예시 |
| -------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| license:LICENSE_KEYWORD
| [**license:apache-2.0**](https://github.com/search?utf8=%E2%9C%93&q=license%3Aapache-2.0&type=Repositories&ref=searchresults) matches repositories that are licensed under Apache License 2.0. |
-### Search by public or private repository
+{% endif %}
+
+### Search by repository visibility
-You can filter your search based on whether a repository is public or private.
+You can filter your search based on the visibility of the repositories. For more information, see "[About repository visibility](/github/creating-cloning-and-archiving-repositories/about-repository-visibility)."
-| Qualifier | 예시 |
-| ------------ | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
-| `is:public` | [**is:public org:github**](https://github.com/search?q=is%3Apublic+org%3Agithub&type=Repositories&utf8=%E2%9C%93) matches repositories owned by GitHub that are public. |
-| `is:private` | [**is:private pages**](https://github.com/search?utf8=%E2%9C%93&q=pages+is%3Aprivate&type=Repositories) matches private repositories you have access to and that contain the word "pages." |
+| Qualifier | Example | ------------- | ------------- |{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %} | `is:public` | [**is:public org:github**](https://github.com/search?q=is%3Apublic+org%3Agithub&type=Repositories) matches public repositories owned by {% data variables.product.company_short %}.{% endif %} | `is:internal` | [**is:internal test**](https://github.com/search?q=is%3Ainternal+test&type=Repositories) matches internal repositories that you can access and contain the word "test". | `is:private` | [**is:private pages**](https://github.com/search?q=is%3Aprivate+pages&type=Repositories) matches private repositories that you can access and contain the word "pages."
{% if currentVersion == "free-pro-team@latest" %}
### Search based on whether a repository is a mirror
-You can search repositories based on whether or not they're a mirror and are hosted elsewhere. For more information, see "[Finding ways to contribute to open source on {% data variables.product.prodname_dotcom %}](/github/getting-started-with-github/finding-ways-to-contribute-to-open-source-on-github)."
+You can search repositories based on whether the repositories are mirrors and hosted elsewhere. For more information, see "[Finding ways to contribute to open source on {% data variables.product.prodname_dotcom %}](/github/getting-started-with-github/finding-ways-to-contribute-to-open-source-on-github)."
| Qualifier | 예시 |
| -------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -158,7 +159,7 @@ You can search repositories based on whether or not they're a mirror and are hos
### Search based on whether a repository is archived
-You can search repositories based on whether or not they're [archived](/articles/about-archiving-repositories).
+You can search repositories based on whether or not the repositories are archived. For more information, see "[About archiving repositories](/github/creating-cloning-and-archiving-repositories/about-archiving-repositories)."
| Qualifier | 예시 |
| ---------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -166,6 +167,7 @@ You can search repositories based on whether or not they're [archived](/articles
| `archived:false` | [**archived:false GNOME**](https://github.com/search?utf8=%E2%9C%93&q=archived%3Afalse+GNOME&type=) matches repositories that are not archived and contain the word "GNOME." |
{% if currentVersion == "free-pro-team@latest" %}
+
### Search based on number of issues with `good first issue` or `help wanted` labels
You can search for repositories that have a minimum number of issues labeled `help-wanted` or `good-first-issue` with the qualifiers `help-wanted-issues:>n` and `good-first-issues:>n`. For more information, see "[Encouraging helpful contributions to your project with labels](/github/building-a-strong-community/encouraging-helpful-contributions-to-your-project-with-labels)."
@@ -174,6 +176,7 @@ You can search for repositories that have a minimum number of issues labeled `he
| -------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
| `good-first-issues:>n` | [**good-first-issues:>2 javascript**](https://github.com/search?utf8=%E2%9C%93&q=javascript+good-first-issues%3A%3E2&type=) matches repositories with more than two issues labeled `good-first-issue` and that contain the word "javascript." |
| `help-wanted-issues:>n` | [**help-wanted-issues:>4 react**](https://github.com/search?utf8=%E2%9C%93&q=react+help-wanted-issues%3A%3E4&type=) matches repositories with more than four issues labeled `help-wanted` and that contain the word "React." |
+
{% endif %}
### 더 읽을거리
diff --git a/translations/ko-KR/content/github/searching-for-information-on-github/searching-issues-and-pull-requests.md b/translations/ko-KR/content/github/searching-for-information-on-github/searching-issues-and-pull-requests.md
index 4165e9742be2..b10689f90fbc 100644
--- a/translations/ko-KR/content/github/searching-for-information-on-github/searching-issues-and-pull-requests.md
+++ b/translations/ko-KR/content/github/searching-for-information-on-github/searching-issues-and-pull-requests.md
@@ -64,14 +64,11 @@ You can filter issues and pull requests based on whether they're open or closed
| `is:open` | [**performance is:open is:issue**](https://github.com/search?q=performance+is%3Aopen+is%3Aissue&type=Issues) matches open issues with the word "performance." |
| `is:closed` | [**android is:closed**](https://github.com/search?utf8=%E2%9C%93&q=android+is%3Aclosed&type=) matches closed issues and pull requests with the word "android." |
-### Search by public or private repository
+### Filter by repository visibility
-If you're [searching across all of {% data variables.product.product_name %}](https://github.com/search), it can be helpful to filter your results based on whether the repository is public or private. You can do this with `is:public` and `is:private`.
+You can filter by the visibility of the repository containing the issues and pull requests using the `is` qualifier. For more information, see "[About repository visibility](/github/creating-cloning-and-archiving-repositories/about-repository-visibility)."
-| Qualifier | 예시 |
-| ------------ | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `is:public` | [**is:public**](https://github.com/search?q=is%3Apublic&type=Issues) matches issues and pull requests in all public repositories. |
-| `is:private` | [**is:private cupcake**](https://github.com/search?q=is%3Aprivate&type=Issues) matches issues and pull requests that contain the word "cupcake" in private repositories you have access to. |
+| Qualifier | Example | ------------- | ------------- |{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %} | `is:public` | [**is:public**](https://github.com/search?q=is%3Apublic&type=Issues) matches issues and pull requests in public repositories.{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.20" or currentVersion == "github-ae@latest" %} | `is:internal` | [**is:internal**](https://github.com/search?q=is%3Ainternal&type=Issues) matches issues and pull requests in internal repositories.{% endif %} | `is:private` | [**is:private cupcake**](https://github.com/search?q=is%3Aprivate+cupcake&type=Issues) matches issues and pull requests that contain the word "cupcake" in private repositories you can access.
### Search by author
diff --git a/translations/ko-KR/content/github/setting-up-and-managing-organizations-and-teams/about-your-organization-dashboard.md b/translations/ko-KR/content/github/setting-up-and-managing-organizations-and-teams/about-your-organization-dashboard.md
index 4182fd11b568..fe9be4889c25 100644
--- a/translations/ko-KR/content/github/setting-up-and-managing-organizations-and-teams/about-your-organization-dashboard.md
+++ b/translations/ko-KR/content/github/setting-up-and-managing-organizations-and-teams/about-your-organization-dashboard.md
@@ -37,8 +37,8 @@ For instance, the organization news feed shows updates when someone in the organ
- Submits a pull request review comment.
- Forks a repository.
- Creates a wiki page.
- - Pushes commits.
- - Creates a public repository.
+ - Pushes commits.{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+ - Creates a public repository.{% endif %}
### Further information
diff --git a/translations/ko-KR/content/github/setting-up-and-managing-organizations-and-teams/restricting-repository-visibility-changes-in-your-organization.md b/translations/ko-KR/content/github/setting-up-and-managing-organizations-and-teams/restricting-repository-visibility-changes-in-your-organization.md
index bbb35db9fdf4..d2cf31b2122f 100644
--- a/translations/ko-KR/content/github/setting-up-and-managing-organizations-and-teams/restricting-repository-visibility-changes-in-your-organization.md
+++ b/translations/ko-KR/content/github/setting-up-and-managing-organizations-and-teams/restricting-repository-visibility-changes-in-your-organization.md
@@ -9,7 +9,7 @@ versions:
github-ae: '*'
---
-You can restrict the ability to change repository visibility to organization owners only, or allow members with admin privileges for a repository to also change visibility from private to public or public to private.
+You can restrict the ability to change repository visibility to organization owners only, or allow members with admin privileges for a repository to also change visibility.
{% warning %}
@@ -24,3 +24,7 @@ You can restrict the ability to change repository visibility to organization own
{% data reusables.organizations.member-privileges %}
5. Under "Repository visibility change", deselect **Allow members to change repository visibilities for this organization**. 
6. Click **Save**.
+
+### 더 읽을거리
+
+- "[About repository visibility](/github/creating-cloning-and-archiving-repositories/about-repository-visibility)"
diff --git a/translations/ko-KR/content/github/setting-up-and-managing-organizations-and-teams/reviewing-the-audit-log-for-your-organization.md b/translations/ko-KR/content/github/setting-up-and-managing-organizations-and-teams/reviewing-the-audit-log-for-your-organization.md
index cd5421ea484e..7a37fcd35f80 100644
--- a/translations/ko-KR/content/github/setting-up-and-managing-organizations-and-teams/reviewing-the-audit-log-for-your-organization.md
+++ b/translations/ko-KR/content/github/setting-up-and-managing-organizations-and-teams/reviewing-the-audit-log-for-your-organization.md
@@ -1,6 +1,7 @@
---
title: Reviewing the audit log for your organization
intro: 'The audit log allows organization admins to quickly review the actions performed by members of your organization. It includes details such as who performed the action, what the action was, and when it was performed.'
+miniTocMaxHeadingLevel: 4
redirect_from:
- /articles/reviewing-the-audit-log-for-your-organization
versions:
@@ -11,7 +12,7 @@ versions:
### Accessing the audit log
-The audit log lists actions performed within the last 90 days. Only owners can access an organization's audit log.
+The audit log lists events triggered by activities that affect your organization within the last 90 days. Only owners can access an organization's audit log.
{% data reusables.profile.access_profile %}
{% data reusables.profile.access_org %}
@@ -26,73 +27,111 @@ The audit log lists actions performed within the last 90 days. Only owners can a
To search for specific events, use the `action` qualifier in your query. Actions listed in the audit log are grouped within the following categories:
-| Category Name | 설명 |
-| ------------------------------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |{% if currentVersion == "free-pro-team@latest" %}
-| `계정` | Contains all activities related to your organization account.{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-| `결제` | Contains all activities related to your organization's billing.{% endif %}
-| `discussion_post` | Contains all activities related to discussions posted to a team page. |
-| `discussion_post_reply` | Contains all activities related to replies to discussions posted to a team page. |
-| `후크` | Contains all activities related to webhooks. |
-| `integration_installation_request` | Contains all activities related to organization member requests for owners to approve integrations for use in the organization. |{% if currentVersion == "free-pro-team@latest" %}
-| `marketplace_agreement_signature` | Contains all activities related to signing the {% data variables.product.prodname_marketplace %} Developer Agreement. |
-| `marketplace_listing` | Contains all activities related to listing apps in {% data variables.product.prodname_marketplace %}.{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
-| `members_can_create_pages` | Contains all activities related to disabling the publication of {% data variables.product.prodname_pages %} sites for repositories in the organization. For more information, see "[Restricting publication of {% data variables.product.prodname_pages %} sites for your organization](/github/setting-up-and-managing-organizations-and-teams/disabling-publication-of-github-pages-sites-for-your-organization)." |{% endif %}
-| `org` | Contains all activities related to organization membership{% if currentVersion == "free-pro-team@latest" %}
-| `org_credential_authorization` | Contains all activities related to authorizing credentials for use with SAML single sign-on.{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "github-ae@latest" %}
-| `organization_label` | Contains all activities related to default labels for repositories in your organization.{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-| `payment_method` | Contains all activities related to how your organization pays for GitHub.{% endif %}
-| `profile_picture` | Contains all activities related to your organization's profile picture. |
-| `프로젝트` | Contains all activities related to project boards. |
-| `protected_branch` | Contains all activities related to protected branches. |
-| `repo` | Contains all activities related to the repositories owned by your organization.{% if currentVersion == "free-pro-team@latest" %}
-| `repository_content_analysis` | Contains all activities related to [enabling or disabling data use for a private repository](/articles/about-github-s-use-of-your-data). |
-| `repository_dependency_graph` | Contains all activities related to [enabling or disabling the dependency graph for a private repository](/github/visualizing-repository-data-with-graphs/exploring-the-dependencies-and-dependents-of-a-repository).{% endif %}{% if currentVersion != "github-ae@latest" %}
-| `repository_vulnerability_alert` | Contains all activities related to [{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot %}{% else %}security{% endif %} alerts for vulnerable dependencies](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies).{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-| `sponsors` | Contains all events related to sponsor buttons (see "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)"){% endif %}{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-| `팀` | Contains all activities related to teams in your organization.{% endif %}
-| `team_discussions` | Contains activities related to managing team discussions for an organization. |
+| Category name | 설명 |
+| ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |{% if currentVersion == "free-pro-team@latest" %}
+| [`계정`](#account-category-actions) | Contains all activities related to your organization account. |
+| [`advisory_credit`](#advisory_credit-category-actions) | Contains all activities related to crediting a contributor for a security advisory in the {% data variables.product.prodname_advisory_database %}. For more information, see "[About {% data variables.product.prodname_dotcom %} Security Advisories](/github/managing-security-vulnerabilities/about-github-security-advisories)." |
+| [`결제`](#billing-category-actions) | Contains all activities related to your organization's billing. |
+| [`dependabot_alerts`](#dependabot_alerts-category-actions) | Contains organization-level configuration activities for {% data variables.product.prodname_dependabot %} alerts in existing repositories. For more information, see "[About alerts for vulnerable dependencies](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies)." |
+| [`dependabot_alerts_new_repos`](#dependabot_alerts_new_repos-category-actions) | Contains organization-level configuration activities for {% data variables.product.prodname_dependabot %} alerts in new repositories created in the organization. |
+| [`dependabot_security_updates`](#dependabot_security_updates-category-actions) | Contains organization-level configuration activities for {% data variables.product.prodname_dependabot_security_updates %} in existing repositories. For more information, see "[Configuring {% data variables.product.prodname_dependabot_security_updates %}](/github/managing-security-vulnerabilities/configuring-dependabot-security-updates)." |
+| [`dependabot_security_updates_new_repos`](#dependabot_security_updates_new_repos-category-actions) | Contains organization-level configuration activities for {% data variables.product.prodname_dependabot_security_updates %} for new repositories created in the organization. |
+| [`dependency_graph`](#dependency_graph-category-actions) | Contains organization-level configuration activities for dependency graphs for repositories. For more information, see "[About the dependency graph](/github/visualizing-repository-data-with-graphs/about-the-dependency-graph)." |
+| [`dependency_graph_new_repos`](#dependency_graph_new_repos-category-actions) | Contains organization-level configuration activities for new repositories created in the organization.{% endif %}
+| [`discussion_post`](#discussion_post-category-actions) | Contains all activities related to discussions posted to a team page. |
+| [`discussion_post_reply`](#discussion_post_reply-category-actions) | Contains all activities related to replies to discussions posted to a team page. |
+| [`후크`](#hook-category-actions) | Contains all activities related to webhooks. |
+| [`integration_installation_request`](#integration_installation_request-category-actions) | Contains all activities related to organization member requests for owners to approve integrations for use in the organization. |
+| [`이슈`](#issue-category-actions) | Contains activities related to deleting an issue. |{% if currentVersion == "free-pro-team@latest" %}
+| [`marketplace_agreement_signature`](#marketplace_agreement_signature-category-actions) | Contains all activities related to signing the {% data variables.product.prodname_marketplace %} Developer Agreement. |
+| [`marketplace_listing`](#marketplace_listing-category-actions) | Contains all activities related to listing apps in {% data variables.product.prodname_marketplace %}.{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
+| [`members_can_create_pages`](#members_can_create_pages-category-actions) | Contains all activities related to disabling the publication of {% data variables.product.prodname_pages %} sites for repositories in the organization. For more information, see "[Restricting publication of {% data variables.product.prodname_pages %} sites for your organization](/github/setting-up-and-managing-organizations-and-teams/disabling-publication-of-github-pages-sites-for-your-organization)." |{% endif %}
+| [`org`](#org-category-actions) | Contains activities related to organization membership.{% if currentVersion == "free-pro-team@latest" %}
+| [`org_credential_authorization`](#org_credential_authorization-category-actions) | Contains all activities related to authorizing credentials for use with SAML single sign-on.{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "github-ae@latest" %}
+| [`organization_label`](#organization_label-category-actions) | Contains all activities related to default labels for repositories in your organization.{% endif %}
+| [`oauth_application`](#oauth_application-category-actions) | Contains all activities related to OAuth Apps. |{% if currentVersion == "free-pro-team@latest" %}
+| [`payment_method`](#payment_method-category-actions) | Contains all activities related to how your organization pays for GitHub.{% endif %}
+| [`profile_picture`](#profile_picture-category-actions) | Contains all activities related to your organization's profile picture. |
+| [`프로젝트`](#project-category-actions) | Contains all activities related to project boards. |
+| [`protected_branch`](#protected_branch-category-actions) | Contains all activities related to protected branches. |
+| [`repo`](#repo-category-actions) | Contains activities related to the repositories owned by your organization.{% if currentVersion == "free-pro-team@latest" %}
+| [`repository_advisory`](#repository_advisory-category-actions) | Contains repository-level activities related to security advisories in the {% data variables.product.prodname_advisory_database %}. For more information, see "[About {% data variables.product.prodname_dotcom %} Security Advisories](/github/managing-security-vulnerabilities/about-github-security-advisories)." |
+| [`repository_content_analysis`](#repository_content_analysis-category-actions) | Contains all activities related to [enabling or disabling data use for a private repository](/articles/about-github-s-use-of-your-data).{% endif %}{% if currentVersion != "github-ae@latest" %}
+| [`repository_dependency_graph`](#repository_dependency_graph-category-actions) | Contains repository-level activities related to enabling or disabling the dependency graph for a |
+| {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repository. For more information, see "[About the dependency graph](/github/visualizing-repository-data-with-graphs/about-the-dependency-graph)."{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %} | |
+| [`repository_secret_scanning`](#repository_secret_scanning-category-actions) | Contains repository-level activities related to secret scanning. For more information, see "[About secret scanning](/github/administering-a-repository/about-secret-scanning)." |{% endif %}{% if currentVersion != "github-ae@latest" %}
+| [`repository_vulnerability_alert`](#repository_vulnerability_alert-category-actions) | Contains all activities related to [{% data variables.product.prodname_dependabot_alerts %} for vulnerable dependencies](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies).{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| [`repository_vulnerability_alerts`](#repository_vulnerability_alerts-category-actions) | Contains repository-level configuration activities for {% data variables.product.prodname_dependabot %} alerts. |{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %}
+| [`secret_scanning`](#secret_scanning-category-actions) | Contains organization-level configuration activities for secret scanning in existing repositories. For more information, see "[About secret scanning](/github/administering-a-repository/about-secret-scanning)." |
+| [`secret_scanning_new_repos`](#secret_scanning_new_repos-category-actions) | Contains organization-level configuration activities for secret scanning for new repositories created in the organization. |{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| [`sponsors`](#sponsors-category-actions) | Contains all events related to sponsor buttons (see "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)"){% endif %}{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
+| [`팀`](#team-category-actions) | Contains all activities related to teams in your organization.{% endif %}
+| [`team_discussions`](#team_discussions-category-actions) | Contains activities related to managing team discussions for an organization. |
You can search for specific sets of actions using these terms. 예시:
* `action:team` finds all events grouped within the team category.
* `-action:hook` excludes all events in the webhook category.
-Each category has a set of associated events that you can filter on. 예시:
+Each category has a set of associated actions that you can filter on. 예시:
* `action:team.create` finds all events where a team was created.
* `-action:hook.events_changed` excludes all events where the events on a webhook have been altered.
-This list describes the available categories and associated events:
-
-{% if currentVersion == "free-pro-team@latest" %}- [The `account` category](#the-account-category)
-- [The `billing` category](#the-billing-category){% endif %}
-- [The `discussion_post` category](#the-discussion_post-category)
-- [The `discussion_post_reply` category](#the-discussion_post_reply-category)
-- [The `hook` category](#the-hook-category)
-- [The `integration_installation_request` category](#the-integration_installation_request-category)
-- [The `issue` category](#the-issue-category){% if currentVersion == "free-pro-team@latest" %}
-- [The `marketplace_agreement_signature` category](#the-marketplace_agreement_signature-category)
-- [The `marketplace_listing` category](#the-marketplace_listing-category){% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
-- [The `members_can_create_pages` category](#the-members_can_create_pages-category){% endif %}
-- [The `org` category](#the-org-category){% if currentVersion == "free-pro-team@latest" %}
-- [The `org_credential_authorization` category](#the-org_credential_authorization-category){% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "github-ae@latest" %}
-- [The `organization_label` category](#the-organization_label-category){% endif %}
-- [The `oauth_application` category](#the-oauth_application-category){% if currentVersion == "free-pro-team@latest" %}
-- [The `payment_method` category](#the-payment_method-category){% endif %}
-- [The `profile_picture` category](#the-profile_picture-category)
-- [The `project` category](#the-project-category)
-- [The `protected_branch` category](#the-protected_branch-category)
-- [The `repo` category](#the-repo-category){% if currentVersion == "free-pro-team@latest" %}
-- [The `repository_content_analysis` category](#the-repository_content_analysis-category)
-- [The `repository_dependency_graph` category](#the-repository_dependency_graph-category){% endif %}{% if currentVersion != "github-ae@latest" %}
-- [The `repository_vulnerability_alert` category](#the-repository_vulnerability_alert-category){% endif %}{% if currentVersion == "free-pro-team@latest" %}
-- [The `sponsors` category](#the-sponsors-category){% endif %}{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-- [The `team` category](#the-team-category){% endif %}
-- [The `team_discussions` category](#the-team_discussions-category)
+#### Search based on time of action
+
+Use the `created` qualifier to filter events in the audit log based on when they occurred. {% data reusables.time_date.date_format %} {% data reusables.time_date.time_format %}
+
+{% data reusables.search.date_gt_lt %} For example:
+
+ * `created:2014-07-08` finds all events that occurred on July 8th, 2014.
+ * `created:>=2014-07-08` finds all events that occurred on or after July 8th, 2014.
+ * `created:<=2014-07-08` finds all events that occurred on or before July 8th, 2014.
+ * `created:2014-07-01..2014-07-31` finds all events that occurred in the month of July 2014.
+
+The audit log contains data for the past 90 days, but you can use the `created` qualifier to search for events earlier than that.
+
+#### Search based on location
+
+Using the qualifier `country`, you can filter events in the audit log based on the originating country. You can use a country's two-letter short code or its full name. Keep in mind that countries with spaces in their name will need to be wrapped in quotation marks. 예시:
+
+ * `country:de` finds all events that occurred in Germany.
+ * `country:Mexico` finds all events that occurred in Mexico.
+ * `country:"United States"` all finds events that occurred in the United States.
+
+{% if currentVersion == "free-pro-team@latest" %}
+### Exporting the audit log
+
+{% data reusables.audit_log.export-log %}
+{% data reusables.audit_log.exported-log-keys-and-values %}
+{% endif %}
+
+### Using the Audit log API
+
+{% note %}
+
+**Note**: The Audit log API is available for organizations using {% data variables.product.prodname_enterprise %}. {% data reusables.gated-features.more-info-org-products %}
+
+{% endnote %}
+
+To ensure a secure IP and maintain compliance for your organization, you can use the Audit log API to keep copies of your audit log data and monitor:
+* Access to your organization or repository settings.
+* Changes in permissions.
+* Added or removed users in an organization, repository, or team.
+* Users being promoted to admin.
+* Changes to permissions of a GitHub App.
+
+The GraphQL response can include data for up to 90 to 120 days.
+
+For example, you can make a GraphQL request to see all the new organization members added to your organization. For more information, see the "[GraphQL API Audit Log](/graphql/reference/interfaces#auditentry/)."
+
+### Audit log actions
+
+An overview of some of the most common actions that are recorded as events in the audit log.
{% if currentVersion == "free-pro-team@latest" %}
-##### The `account` category
+#### `account` category actions
| 동작 | 설명 |
| ----------------------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -101,30 +140,81 @@ This list describes the available categories and associated events:
| `pending_plan_change` | Triggered when an organization owner or billing manager [cancels or downgrades a paid subscription](/articles/how-does-upgrading-or-downgrading-affect-the-billing-process/). |
| `pending_subscription_change` | Triggered when a [{% data variables.product.prodname_marketplace %} free trial starts or expires](/articles/about-billing-for-github-marketplace/). |
-##### The `billing` category
+#### `advisory_credit` category actions
+
+| 동작 | 설명 |
+| --------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| `accept` | Triggered when someone accepts credit for a security advisory. For more information, see "[Editing a security advisory](/github/managing-security-vulnerabilities/editing-a-security-advisory)." |
+| `create` | Triggered when the administrator of a security advisory adds someone to the credit section. |
+| `decline` | Triggered when someone declines credit for a security advisory. |
+| `destroy` | Triggered when the administrator of a security advisory removes someone from the credit section. |
+
+#### `billing` category actions
| 동작 | 설명 |
| --------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------ |
| `change_billing_type` | Triggered when your organization [changes how it pays for {% data variables.product.prodname_dotcom %}](/articles/adding-or-editing-a-payment-method). |
| `change_email` | Triggered when your organization's [billing email address](/articles/setting-your-billing-email) changes. |
+#### `dependabot_alerts` category actions
+
+| 동작 | 설명 |
+| ------ | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| `비활성화` | Triggered when an organization owner disables {% data variables.product.prodname_dependabot_alerts %} for all existing {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repositories. For more information, see "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)." |
+| `활성화` | Triggered when an organization owner enables {% data variables.product.prodname_dependabot_alerts %} for all existing {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repositories. |
+
+#### `dependabot_alerts_new_repos` category actions
+
+| 동작 | 설명 |
+| ------ | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `비활성화` | Triggered when an organization owner disables {% data variables.product.prodname_dependabot_alerts %} for all new {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repositories. For more information, see "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)." |
+| `활성화` | Triggered when an organization owner enbles {% data variables.product.prodname_dependabot_alerts %} for all new {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repositories. |
+
+#### `dependabot_security_updates` category actions
+
+| 동작 | 설명 |
+| ------ | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `비활성화` | Triggered when an organization owner disables {% data variables.product.prodname_dependabot_security_updates %} for all existing repositories. For more information, see "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)." |
+| `활성화` | Triggered when an organization owner enables {% data variables.product.prodname_dependabot_security_updates %} for all existing repositories. |
+
+#### `dependabot_security_updates_new_repos` category actions
+
+| 동작 | 설명 |
+| ------ | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `비활성화` | Triggered when an organization owner disables {% data variables.product.prodname_dependabot_security_updates %} for all new repositories. For more information, see "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)." |
+| `활성화` | Triggered when an organization owner enables {% data variables.product.prodname_dependabot_security_updates %} for all new repositories. |
+
+#### `dependency_graph` category actions
+
+| 동작 | 설명 |
+| ------ | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `비활성화` | Triggered when an organization owner disables the dependency graph for all existing repositories. For more information, see "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)." |
+| `활성화` | Triggered when an organization owner enables the dependency graph for all existing repositories. |
+
+#### `dependency_graph_new_repos` category actions
+
+| 동작 | 설명 |
+| ------ | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `비활성화` | Triggered when an organization owner disables the dependency graph for all new repositories. For more information, see "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)." |
+| `활성화` | Triggered when an organization owner enables the dependency graph for all new repositories. |
+
{% endif %}
-##### The `discussion_post` category
+#### `discussion_post` category actions
| 동작 | 설명 |
| --------- | --------------------------------------------------------------------------------------------------------------- |
| `업데이트` | Triggered when [a team discussion post is edited](/articles/managing-disruptive-comments/#editing-a-comment). |
| `destroy` | Triggered when [a team discussion post is deleted](/articles/managing-disruptive-comments/#deleting-a-comment). |
-##### The `discussion_post_reply` category
+#### `discussion_post_reply` category actions
| 동작 | 설명 |
| --------- | -------------------------------------------------------------------------------------------------------------------------- |
| `업데이트` | Triggered when [a reply to a team discussion post is edited](/articles/managing-disruptive-comments/#editing-a-comment). |
| `destroy` | Triggered when [a reply to a team discussion post is deleted](/articles/managing-disruptive-comments/#deleting-a-comment). |
-##### The `hook` category
+#### `hook` category actions
| 동작 | 설명 |
| ---------------- | -------------------------------------------------------------------------------------------------------------- |
@@ -133,14 +223,14 @@ This list describes the available categories and associated events:
| `destroy` | Triggered when an existing hook was removed from a repository. |
| `events_changed` | Triggered when the events on a hook have been altered. |
-##### The `integration_installation_request` category
+#### `integration_installation_request` category actions
| 동작 | 설명 |
| -------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| `create` | Triggered when an organization member requests that an organization owner install an integration for use in the organization. |
| `close` | Triggered when a request to install an integration for use in an organization is either approved or denied by an organization owner, or canceled by the organization member who opened the request. |
-##### The `issue` category
+#### `issue` category actions
| 동작 | 설명 |
| --------- | ---------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -148,13 +238,13 @@ This list describes the available categories and associated events:
{% if currentVersion == "free-pro-team@latest" %}
-##### The `marketplace_agreement_signature` category
+#### `marketplace_agreement_signature` category actions
| 동작 | 설명 |
| -------- | -------------------------------------------------------------------------------------------------- |
| `create` | Triggered when you sign the {% data variables.product.prodname_marketplace %} Developer Agreement. |
-##### The `marketplace_listing` category
+#### `marketplace_listing` category actions
| 동작 | 설명 |
| --------- | --------------------------------------------------------------------------------------------------------------- |
@@ -168,7 +258,7 @@ This list describes the available categories and associated events:
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
-##### The `members_can_create_pages` category
+#### `members_can_create_pages` category actions
For more information, see "[Restricting publication of {% data variables.product.prodname_pages %} sites for your organization](/github/setting-up-and-managing-organizations-and-teams/disabling-publication-of-github-pages-sites-for-your-organization)."
@@ -179,7 +269,7 @@ For more information, see "[Restricting publication of {% data variables.product
{% endif %}
-##### The `org` category
+#### `org` category actions
| 동작 | 설명 |
| -------------------------------------------------------------------------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |{% if currentVersion == "free-pro-team@latest"%}
@@ -222,7 +312,7 @@ For more information, see "[Restricting publication of {% data variables.product
| `update_terms_of_service` | Triggered when an organization changes between the Standard Terms of Service and the Corporate Terms of Service. For more information, see "[Upgrading to the Corporate Terms of Service](/articles/upgrading-to-the-corporate-terms-of-service)."{% endif %}
{% if currentVersion == "free-pro-team@latest" %}
-##### The `org_credential_authorization` category
+#### `org_credential_authorization` category actions
| 동작 | 설명 |
| -------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -233,7 +323,7 @@ For more information, see "[Restricting publication of {% data variables.product
{% endif %}
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "github-ae@latest" %}
-##### The `organization_label` category
+#### `organization_label` category actions
| 동작 | 설명 |
| --------- | ------------------------------------------ |
@@ -243,7 +333,7 @@ For more information, see "[Restricting publication of {% data variables.product
{% endif %}
-##### The `oauth_application` category
+#### `oauth_application` category actions
| 동작 | 설명 |
| --------------- | ------------------------------------------------------------------------------------------------------------------ |
@@ -255,7 +345,7 @@ For more information, see "[Restricting publication of {% data variables.product
{% if currentVersion == "free-pro-team@latest" %}
-##### The `payment_method` category
+#### `payment_method` category actions
| 동작 | 설명 |
| -------- | ------------------------------------------------------------------------------------------ |
@@ -265,12 +355,12 @@ For more information, see "[Restricting publication of {% data variables.product
{% endif %}
-##### The `profile_picture` category
+#### `profile_picture` category actions
| 동작 | 설명 |
| ---- | --------------------------------------------------------------------- |
| 업데이트 | Triggered when you set or update your organization's profile picture. |
-##### The `project` category
+#### `project` category actions
| 동작 | 설명 |
| ------------------------ | ------------------------------------------------------------------------------------------------------------------------------------------------ |
@@ -284,7 +374,7 @@ For more information, see "[Restricting publication of {% data variables.product
| `update_team_permission` | Triggered when a team's project board permission level is changed or when a team is added or removed from a project board. |
| `update_user_permission` | Triggered when an organization member or outside collaborator is added to or removed from a project board or has their permission level changed. |
-##### The `protected_branch` category
+#### `protected_branch` category actions
| 동작 | 설명 |
| ----------------------------------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -304,14 +394,14 @@ For more information, see "[Restricting publication of {% data variables.product
| `update_linear_history_requirement_enforcement_level` | Triggered when required linear commit history is enabled or disabled for a protected branch. |
{% endif %}
-##### The `repo` category
+#### `repo` category actions
| 동작 | 설명 |
| ------------------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `액세스` | Triggered when a repository owned by an organization is [switched from "private" to "public"](/articles/making-a-private-repository-public) (or vice versa). |
+| `액세스` | Triggered when a user [changes the visibility](/github/administering-a-repository/setting-repository-visibility) of a repository in the organization. |
| `add_member` | Triggered when a user accepts an [invitation to have collaboration access to a repository](/articles/inviting-collaborators-to-a-personal-repository). |
| `add_topic` | Triggered when a repository admin [adds a topic](/articles/classifying-your-repository-with-topics) to a repository. |
-| `archived` | Triggered when a repository admin [archives a repository](/articles/about-archiving-repositories).{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
+| `archived` | Triggered when a repository admin [archives a repository](/articles/about-archiving-repositories).{% if enterpriseServerVersions contains currentVersion %}
| `config.disable_anonymous_git_access` | Triggered when [anonymous Git read access is disabled](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository) in a public repository. |
| `config.enable_anonymous_git_access` | Triggered when [anonymous Git read access is enabled](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository) in a public repository. |
| `config.lock_anonymous_git_access` | Triggered when a repository's [anonymous Git read access setting is locked](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access). |
@@ -334,35 +424,80 @@ For more information, see "[Restricting publication of {% data variables.product
{% if currentVersion == "free-pro-team@latest" %}
-##### The `repository_content_analysis` category
+#### `repository_advisory` category actions
+
+| 동작 | 설명 |
+| ------------------ | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| `close` | Triggered when someone closes a security advisory. For more information, see "[About {% data variables.product.prodname_dotcom %} Security Advisories](/github/managing-security-vulnerabilities/about-github-security-advisories)." |
+| `cve_request` | Triggered when someone requests a CVE (Common Vulnerabilities and Exposures) number from {% data.variables.product.prodname_dotcom %} for a draft security advisory. |
+| `github_broadcast` | Triggered when {% data.variables.product.prodname_dotcom %} makes a security advisory public in the {% data variables.product.prodname_advisory_database %}. |
+| `github_withdraw` | Triggered when {% data.variables.product.prodname_dotcom %} withdraws a security advisory that was published in error. |
+| `open` | Triggered when someone opens a draft security advisory. |
+| `publish` | Triggered when someone publishes a security advisory. |
+| `reopen` | Triggered when someone reopens as draft security advisory. |
+| `업데이트` | Triggered when someone edits a draft or published security advisory. |
+
+#### `repository_content_analysis` category actions
| 동작 | 설명 |
| ------ | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| `활성화` | Triggered when an organization owner or person with admin access to the repository [enables data use settings for a private repository](/github/understanding-how-github-uses-and-protects-your-data/managing-data-use-settings-for-your-private-repository). |
| `비활성화` | Triggered when an organization owner or person with admin access to the repository [disables data use settings for a private repository](/github/understanding-how-github-uses-and-protects-your-data/managing-data-use-settings-for-your-private-repository). |
-##### The `repository_dependency_graph` category
+{% endif %}{% if currentVersion != "github-ae@latest" %}
-| 동작 | 설명 |
-| ------ | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `활성화` | Triggered when a repository owner or person with admin access to the repository [enables the dependency graph for a private repository](/github/visualizing-repository-data-with-graphs/exploring-the-dependencies-and-dependents-of-a-repository). |
-| `비활성화` | Triggered when a repository owner or person with admin access to the repository [disables the dependency graph for a private repository](/github/visualizing-repository-data-with-graphs/exploring-the-dependencies-and-dependents-of-a-repository). |
+#### `repository_dependency_graph` category actions
+
+| 동작 | 설명 |
+| ------ | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `비활성화` | Triggered when a repository owner or person with admin access to the repository disables the dependency graph for a {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repository. For more information, see "[About the dependency graph](/github/visualizing-repository-data-with-graphs/about-the-dependency-graph)." |
+| `활성화` | Triggered when a repository owner or person with admin access to the repository enables the dependency graph for a {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repository. |
+
+{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %}
+#### `repository_secret_scanning` category actions
+
+| 동작 | 설명 |
+| ------ | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `비활성화` | Triggered when a repository owner or person with admin access to the repository disables secret scanning for a {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repository. For more information, see "[About secret scanning](/github/administering-a-repository/about-secret-scanning)." |
+| `활성화` | Triggered when a repository owner or person with admin access to the repository enables secret scanning for a {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repository. |
+
+{% endif %}{% if currentVersion != "github-ae@latest" %}
+#### `repository_vulnerability_alert` category actions
+
+| 동작 | 설명 |
+| -------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `create` | Triggered when {% data variables.product.product_name %} creates a {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot %}{% else %}security{% endif %} alert for a repository that uses a vulnerable dependency. For more information, see "[About alerts for vulnerable dependencies](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies)." |
+| `해제` | Triggered when an organization owner or person with admin access to the repository dismisses a {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot %}{% else %}security{% endif %} alert about a vulnerable dependency. |
+| `해결` | Triggered when someone with write access to a repository pushes changes to update and resolve a vulnerability in a project dependency. |
+
+{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+#### `repository_vulnerability_alerts` category actions
+
+| 동작 | 설명 |
+| ------------------------ | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| `authorized_users_teams` | Triggered when an organization owner or a person with admin permissions to the repository updates the list of people or teams authorized to receive {% data variables.product.prodname_dependabot_alerts %} for vulnerable dependencies in the repository. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-security-alerts)." |
+| `비활성화` | Triggered when a repository owner or person with admin access to the repository disables {% data variables.product.prodname_dependabot_alerts %}. |
+| `활성화` | Triggered when a repository owner or person with admin access to the repository enables {% data variables.product.prodname_dependabot_alerts %}. |
+
+{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %}
+#### `secret_scanning` category actions
+
+| 동작 | 설명 |
+| ------ | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `비활성화` | Triggered when an organization owner disables secret scanning for all existing{% if currentVersion == "free-pro-team@latest" %}, private{% endif %} repositories. For more information, see "[About secret scanning](/github/administering-a-repository/about-secret-scanning)." |
+| `활성화` | Triggered when an organization owner enables secret scanning for all existing{% if currentVersion == "free-pro-team@latest" %}, private{% endif %} repositories. |
+
+#### `secret_scanning_new_repos` category actions
+
+| 동작 | 설명 |
+| ------ | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `비활성화` | Triggered when an organization owner disables secret scanning for all new {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repositories. For more information, see "[About secret scanning](/github/administering-a-repository/about-secret-scanning)." |
+| `활성화` | Triggered when an organization owner enables secret scanning for all new {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repositories. |
-{% endif %}
-{% if currentVersion != "github-ae@latest" %}
-##### The `repository_vulnerability_alert` category
-
-| 동작 | 설명 |
-| ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `create` | Triggered when {% data variables.product.product_name %} creates a [{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot %}{% else %}security{% endif %} alert for a vulnerable dependency](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies) in a particular repository. |
-| `해결` | Triggered when someone with write access to a repository [pushes changes to update and resolve a vulnerability](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies) in a project dependency. |
-| `해제` | Triggered when an organization owner or person with admin access to the repository dismisses a |
-| {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot %}{% else %}security{% endif %} alert about a vulnerable dependency.{% if currentVersion == "free-pro-team@latest" %} | |
-| `authorized_users_teams` | Triggered when an organization owner or a member with admin permissions to the repository [updates the list of people or teams authorized to receive {% data variables.product.prodname_dependabot_alerts %}](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-dependabot-alerts) for vulnerable dependencies in the repository.{% endif %}
{% endif %}
{% if currentVersion == "free-pro-team@latest" %}
-##### The `sponsors` category
+#### `sponsors` category actions
| 동작 | 설명 |
| ----------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -371,7 +506,7 @@ For more information, see "[Restricting publication of {% data variables.product
{% endif %}
{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-##### The `team` category
+#### `team` category actions
| 동작 | 설명 |
| -------------------- | --------------------------------------------------------------------------------------------------------------------------------------- |
@@ -385,60 +520,13 @@ For more information, see "[Restricting publication of {% data variables.product
| `remove_repository` | Triggered when a repository is no longer under a team's control. |
{% endif %}
-##### The `team_discussions` category
+#### `team_discussions` category actions
| 동작 | 설명 |
| ------ | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| `비활성화` | Triggered when an organization owner disables team discussions for an organization. For more information, see "[Disabling team discussions for your organization](/articles/disabling-team-discussions-for-your-organization)." |
| `활성화` | Triggered when an organization owner enables team discussions for an organization. |
-#### Search based on time of action
-
-Use the `created` qualifier to filter actions in the audit log based on when they occurred. {% data reusables.time_date.date_format %} {% data reusables.time_date.time_format %}
-
-{% data reusables.search.date_gt_lt %} For example:
-
- * `created:2014-07-08` finds all events that occurred on July 8th, 2014.
- * `created:>=2014-07-08` finds all events that occurred on or after July 8th, 2014.
- * `created:<=2014-07-08` finds all events that occurred on or before July 8th, 2014.
- * `created:2014-07-01..2014-07-31` finds all events that occurred in the month of July 2014.
-
-The audit log contains data for the past 90 days, but you can use the `created` qualifier to search for events earlier than that.
-
-#### Search based on location
-
-Using the qualifier `country`, you can filter actions in the audit log based on the originating country. You can use a country's two-letter short code or its full name. Keep in mind that countries with spaces in their name will need to be wrapped in quotation marks. 예시:
-
- * `country:de` finds all events that occurred in Germany.
- * `country:Mexico` finds all events that occurred in Mexico.
- * `country:"United States"` all finds events that occurred in the United States.
-
-{% if currentVersion == "free-pro-team@latest" %}
-### Exporting the audit log
-
-{% data reusables.audit_log.export-log %}
-{% data reusables.audit_log.exported-log-keys-and-values %}
-{% endif %}
-
-### Using the Audit log API
-
-{% note %}
-
-**Note**: The Audit log API is available for organizations using {% data variables.product.prodname_enterprise %}. {% data reusables.gated-features.more-info-org-products %}
-
-{% endnote %}
-
-To ensure a secure IP and maintain compliance for your organization, you can use the Audit log API to keep copies of your audit log data and monitor:
-* Access to your organization or repository settings.
-* Changes in permissions.
-* Added or removed users in an organization, repository, or team.
-* Users being promoted to admin.
-* Changes to permissions of a GitHub App.
-
-The GraphQL response can include data for up to 90 to 120 days.
-
-For example, you can make a GraphQL request to see all the new organization members added to your organization. For more information, see the "[GraphQL API Audit Log](/graphql/reference/interfaces#auditentry/)."
-
### 더 읽을거리
- "[Keeping your organization secure](/articles/keeping-your-organization-secure)"
diff --git a/translations/ko-KR/content/github/setting-up-and-managing-your-enterprise/about-enterprise-accounts.md b/translations/ko-KR/content/github/setting-up-and-managing-your-enterprise/about-enterprise-accounts.md
index bedbb2ace51b..ea38298892b7 100644
--- a/translations/ko-KR/content/github/setting-up-and-managing-your-enterprise/about-enterprise-accounts.md
+++ b/translations/ko-KR/content/github/setting-up-and-managing-your-enterprise/about-enterprise-accounts.md
@@ -20,7 +20,7 @@ An enterprise account allows you to manage multiple {% data variables.product.pr
- Security (single sign-on, two factor authentication)
- Requests and support bundle sharing with {% data variables.contact.enterprise_support %}
-{% data reusables.enterprise-accounts.enterprise-accounts-billing %}
+{% data reusables.enterprise-accounts.enterprise-accounts-billing %} For more information about managing your {% data variables.product.prodname_ghe_cloud %} subscription, see "[Viewing the subscription and usage for your enterprise account](/articles/viewing-the-subscription-and-usage-for-your-enterprise-account)." For more information about managing your {% data variables.product.prodname_ghe_server %} billing settings, see "[Managing billing for your enterprise](/admin/overview/managing-billing-for-your-enterprise)."
For more information about the differences between {% data variables.product.prodname_ghe_cloud %} and {% data variables.product.prodname_ghe_server %}, see "[{% data variables.product.prodname_dotcom %}'s products](/articles/githubs-products)." To upgrade to {% data variables.product.prodname_enterprise %} or to get started with an enterprise account, contact {% data variables.contact.contact_enterprise_sales %}.
diff --git a/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/about-your-profile.md b/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/about-your-profile.md
index ac15d732bfdf..ffdde59eda84 100644
--- a/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/about-your-profile.md
+++ b/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/about-your-profile.md
@@ -13,7 +13,7 @@ versions:
You can add personal information about yourself in your bio, like previous places you've worked, projects you've contributed to, or interests you have that other people may like to know about. For more information, see "[Adding a bio to your profile](/articles/personalizing-your-profile/#adding-a-bio-to-your-profile)."
-{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
{% data reusables.profile.profile-readme %}
@@ -23,19 +23,17 @@ You can add personal information about yourself in your bio, like previous place
People who visit your profile see a timeline of your contribution activity, like issues and pull requests you've opened, commits you've made, and pull requests you've reviewed. You can choose to display only public contributions or to also include private, anonymized contributions. For more information, see "[Viewing contributions on your profile page](/articles/viewing-contributions-on-your-profile-page)" or "[Publicizing or hiding your private contributions on your profile](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)."
-They can also see:
+People who visit your profile can also see the following information.
-- Repositories and gists you own or contribute to. You can showcase your best work by pinning repositories and gists to your profile. For more information, see "[Pinning items to your profile](/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile)."
-- Repositories you've starred. For more information, see "[Saving repositories with stars](/articles/saving-repositories-with-stars/)"
-- An overview of your activity in organizations, repositories, and teams you're most active in. For more information, see "[Showing an overview of your activity on your profile](/articles/showing-an-overview-of-your-activity-on-your-profile).{% if currentVersion == "free-pro-team@latest" %}
-- Badges that advertise your participation in programs like the {% data variables.product.prodname_arctic_vault %}, {% data variables.product.prodname_sponsors %}, or the {% data variables.product.company_short %} Developer Program.
-- If you're using {% data variables.product.prodname_pro %}. For more information, see "[Personalizing your profile](/articles/personalizing-your-profile)."{% endif %}
+- Repositories and gists you own or contribute to. {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}You can showcase your best work by pinning repositories and gists to your profile. For more information, see "[Pinning items to your profile](/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile)."{% endif %}
+- Repositories you've starred. For more information, see "[Saving repositories with stars](/articles/saving-repositories-with-stars/)."
+- An overview of your activity in organizations, repositories, and teams you're most active in. For more information, see "[Showing an overview of your activity on your profile](/articles/showing-an-overview-of-your-activity-on-your-profile)."{% if currentVersion == "free-pro-team@latest" %}
+- Badges that show if you use {% data variables.product.prodname_pro %} or participate in programs like the {% data variables.product.prodname_arctic_vault %}, {% data variables.product.prodname_sponsors %}, or the {% data variables.product.company_short %} Developer Program. For more information, see "[Personalizing your profile](/github/setting-up-and-managing-your-github-profile/personalizing-your-profile#displaying-badges-on-your-profile)."{% endif %}
You can also set a status on your profile to provide information about your availability. For more information, see "[Setting a status](/articles/personalizing-your-profile/#setting-a-status)."
### 더 읽을거리
- "[How do I set up my profile picture?](/articles/how-do-i-set-up-my-profile-picture)"
-- "[Pinning repositories to your profile](/articles/pinning-repositories-to-your-profile)"
- "[Publicizing or hiding your private contributions on your profile](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)"
- "[Viewing contributions on your profile](/articles/viewing-contributions-on-your-profile)"
diff --git a/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme.md b/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme.md
index c29bdd2f5d44..c2733cff0552 100644
--- a/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme.md
+++ b/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme.md
@@ -4,7 +4,6 @@ intro: 'You can add a README to your {% data variables.product.prodname_dotcom %
versions:
free-pro-team: '*'
enterprise-server: '>=2.22'
- github-ae: '*'
---
### About your profile README
diff --git a/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/personalizing-your-profile.md b/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/personalizing-your-profile.md
index fd1a2c778711..573680782235 100644
--- a/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/personalizing-your-profile.md
+++ b/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/personalizing-your-profile.md
@@ -50,9 +50,9 @@ You can change the name that is displayed on your profile. This name may also be
Add a bio to your profile to share information about yourself with other {% data variables.product.product_name %} users. With the help of [@mentions](/articles/basic-writing-and-formatting-syntax) and emoji, you can include information about where you currently or have previously worked, what type of work you do, or even what kind of coffee you drink.
-{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
-For a longer-form and more prominent way of displaying customized information about yourself, you can also use a profile README. For more information on the profile README, see "[Managing your profile README](/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme)."
+For a longer-form and more prominent way of displaying customized information about yourself, you can also use a profile README. For more information, see "[Managing your profile README](/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme)."
{% endif %}
diff --git a/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile.md b/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile.md
index ac5408964463..de4c9ce92b69 100644
--- a/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile.md
+++ b/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile.md
@@ -7,7 +7,6 @@ redirect_from:
versions:
free-pro-team: '*'
enterprise-server: '*'
- github-ae: '*'
---
You can pin a public repository if you own the repository or you've made contributions to the repository. Commits to forks don't count as contributions, so you can't pin a fork that you don't own. For more information, see "[Why are my contributions not showing up on my profile?](/articles/why-are-my-contributions-not-showing-up-on-my-profile)"
diff --git a/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/publicizing-or-hiding-your-private-contributions-on-your-profile.md b/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/publicizing-or-hiding-your-private-contributions-on-your-profile.md
index 4d4f8c1a90c6..099e4aa708e9 100644
--- a/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/publicizing-or-hiding-your-private-contributions-on-your-profile.md
+++ b/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/publicizing-or-hiding-your-private-contributions-on-your-profile.md
@@ -1,6 +1,6 @@
---
title: Publicizing or hiding your private contributions on your profile
-intro: 'Your {% data variables.product.product_name %} profile shows a graph of your repository contributions over the past year. You can choose to show anonymized activity from private repositories in addition to the activity shown from public repositories.'
+intro: 'Your {% data variables.product.product_name %} profile shows a graph of your repository contributions over the past year. You can choose to show anonymized activity from {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" %}private and internal{% else %}private{% endif %} repositories{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %} in addition to the activity from public repositories{% endif %}.'
redirect_from:
- /articles/publicizing-or-hiding-your-private-contributions-on-your-profile
versions:
diff --git a/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/viewing-contributions-on-your-profile.md b/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/viewing-contributions-on-your-profile.md
index 34974346dfe2..747c37af9d8e 100644
--- a/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/viewing-contributions-on-your-profile.md
+++ b/translations/ko-KR/content/github/setting-up-and-managing-your-github-profile/viewing-contributions-on-your-profile.md
@@ -1,6 +1,6 @@
---
title: Viewing contributions on your profile
-intro: 'Your {% data variables.product.product_name %} profile shows off your pinned repositories as well as a graph of your repository contributions over the past year.'
+intro: 'Your {% data variables.product.product_name %} profile shows off {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}your pinned repositories as well as{% endif %} a graph of your repository contributions over the past year.'
redirect_from:
- /articles/viewing-contributions/
- /articles/viewing-contributions-on-your-profile-page/
@@ -11,7 +11,7 @@ versions:
github-ae: '*'
---
-Your contribution graph shows activity from public repositories. You can choose to show activity from both public and private repositories, with specific details of your activity in private repositories anonymized. For more information, see "[Publicizing or hiding your private contributions on your profile](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)."
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}Your contribution graph shows activity from public repositories. {% endif %}You can choose to show activity from {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}both public and{% endif %}private repositories, with specific details of your activity in private repositories anonymized. For more information, see "[Publicizing or hiding your private contributions on your profile](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)."
{% note %}
@@ -33,16 +33,20 @@ On your profile page, certain actions count as contributions:
### Popular repositories
-This section displays your repositories with the most watchers. Once you [pin repositories to your profile](/articles/pinning-repositories-to-your-profile), this section will change to "Pinned repositories."
+This section displays your repositories with the most watchers. {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}Once you [pin repositories to your profile](/articles/pinning-repositories-to-your-profile), this section will change to "Pinned repositories."{% endif %}

+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
### Pinned repositories
This section displays up to six public repositories and can include your repositories as well as repositories you've contributed to. To easily see important details about the repositories you've chosen to feature, each repository in this section includes a summary of the work being done, the number of [stars](/articles/saving-repositories-with-stars/) the repository has received, and the main programming language used in the repository. For more information, see "[Pinning repositories to your profile](/articles/pinning-repositories-to-your-profile)."

+{% endif %}
+
### Contributions calendar
Your contributions calendar shows your contribution activity.
diff --git a/translations/ko-KR/content/github/setting-up-and-managing-your-github-user-account/about-your-personal-dashboard.md b/translations/ko-KR/content/github/setting-up-and-managing-your-github-user-account/about-your-personal-dashboard.md
index 312f0c0909ae..02fc829c1419 100644
--- a/translations/ko-KR/content/github/setting-up-and-managing-your-github-user-account/about-your-personal-dashboard.md
+++ b/translations/ko-KR/content/github/setting-up-and-managing-your-github-user-account/about-your-personal-dashboard.md
@@ -15,7 +15,7 @@ versions:
Your personal dashboard is the first page you'll see when you sign in on {% data variables.product.product_name %}.
-To access your personal dashboard once you're signed in, click the {% octicon "mark-github" aria-label="The github octocat logo" %} in the upper-left corner of any page on {% data variables.product.product_url %}.
+To access your personal dashboard once you're signed in, click the {% octicon "mark-github" aria-label="The github octocat logo" %} in the upper-left corner of any page on {% data variables.product.product_name %}.
### Finding your recent activity
@@ -39,11 +39,11 @@ In the "All activity" section of your news feed, you can view updates from repos
You'll see updates in your news feed when a user you follow:
- Stars a repository.
-- Follows another user.
-- Creates a public repository.
+- Follows another user.{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- Creates a public repository.{% endif %}
- Opens an issue or pull request with "help wanted" or "good first issue" label on a repository you're watching.
-- Pushes commits to a repository you watch.
-- Forks a public repository.
+- Pushes commits to a repository you watch.{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- Forks a public repository.{% endif %}
For more information about starring repositories and following people, see "[Saving repositories with stars](/articles/saving-repositories-with-stars/)" and "[Following people](/articles/following-people)."
diff --git a/translations/ko-KR/content/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository.md b/translations/ko-KR/content/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository.md
index fef0d5774384..c662cd885b67 100644
--- a/translations/ko-KR/content/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository.md
+++ b/translations/ko-KR/content/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository.md
@@ -50,7 +50,7 @@ Repositories owned by an organization can grant more granular access. For more i
### 더 읽을거리
-- "[Permission levels for a user account repository](/articles/permission-levels-for-a-user-account-repository/#collaborator-access-on-a-repository-owned-by-a-user-account)"
+- "[Permission levels for a user account repository](/articles/permission-levels-for-a-user-account-repository/#collaborator-access-for-a-repository-owned-by-a-user-account)"
- "[Removing a collaborator from a personal repository](/articles/removing-a-collaborator-from-a-personal-repository)"
- "[Removing yourself from a collaborator's repository](/articles/removing-yourself-from-a-collaborator-s-repository)"
- "[Organizing members into teams](/articles/organizing-members-into-teams)"
diff --git a/translations/ko-KR/content/github/setting-up-and-managing-your-github-user-account/permission-levels-for-a-user-account-repository.md b/translations/ko-KR/content/github/setting-up-and-managing-your-github-user-account/permission-levels-for-a-user-account-repository.md
index 98fa7963ac5f..661c7281e1e2 100644
--- a/translations/ko-KR/content/github/setting-up-and-managing-your-github-user-account/permission-levels-for-a-user-account-repository.md
+++ b/translations/ko-KR/content/github/setting-up-and-managing-your-github-user-account/permission-levels-for-a-user-account-repository.md
@@ -1,6 +1,6 @@
---
title: Permission levels for a user account repository
-intro: 'A repository owned by a user account has two permission levels: the *repository owner* and *collaborators*.'
+intro: 'A repository owned by a user account has two permission levels: the repository owner and collaborators.'
redirect_from:
- /articles/permission-levels-for-a-user-account-repository
versions:
@@ -9,38 +9,48 @@ versions:
github-ae: '*'
---
+### About permissions levels for a user account repository
+
+Repositories owned by user accounts have one owner. Ownership permissions can't be shared with another user account.
+
+You can also {% if currentVersion == "free-pro-team@latest" %}invite{% else %}add{% endif %} users on {% data variables.product.product_name %} to your repository as collaborators. For more information, see "[Inviting collaborators to a personal repository](/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository)."
+
{% tip %}
-**Tip:** If you require more granular read/write access to a repository owned by your user account, consider transferring the repository to an organization. For more information, see "[Transferring a repository](/articles/transferring-a-repository)."
+**Tip:** If you require more granular access to a repository owned by your user account, consider transferring the repository to an organization. For more information, see "[Transferring a repository](/github/administering-a-repository/transferring-a-repository#transferring-a-repository-owned-by-your-user-account)."
{% endtip %}
-#### Owner access on a repository owned by a user account
-
-The repository owner has full control of the repository. In addition to all the permissions allowed by repository collaborators, the repository owner can:
-
-- {% if currentVersion == "free-pro-team@latest" %}[Invite collaborators](/articles/inviting-collaborators-to-a-personal-repository){% else %}[Add collaborators](/articles/inviting-collaborators-to-a-personal-repository){% endif %}
-- Change the visibility of the repository (from [public to private](/articles/making-a-public-repository-private), or from [private to public](/articles/making-a-private-repository-public)){% if currentVersion == "free-pro-team@latest" %}
-- [Limit interactions with a repository](/articles/limiting-interactions-with-your-repository){% endif %}
-- Merge a pull request on a protected branch, even if there are no approving reviews
-- [Delete the repository](/articles/deleting-a-repository)
-- [Manage a repository's topics](/articles/classifying-your-repository-with-topics){% if currentVersion == "free-pro-team@latest" %}
-- Manage security and analysis settings. For more information, see "[Managing security and analysis settings for your user account](/github/setting-up-and-managing-your-github-user-account/managing-security-and-analysis-settings-for-your-user-account)."{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-- [Enable the dependency graph](/github/visualizing-repository-data-with-graphs/exploring-the-dependencies-and-dependents-of-a-repository) for a private repository{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-- Delete packages. For more information, see "[Deleting a package](/github/managing-packages-with-github-packages/deleting-a-package)."{% endif %}
-- Create and edit repository social cards. For more information, see "[Customizing your repository's social media preview](/articles/customizing-your-repositorys-social-media-preview)."
-- Make the repository a template. For more information, see "[Creating a template repository](/articles/creating-a-template-repository)."{% if currentVersion != "github-ae@latest" %}
-- Receive [{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot_alerts %}{% else %}security alerts{% endif %} for vulnerable dependencies](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies) in a repository.{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-- Dismiss {% data variables.product.prodname_dependabot_alerts %} in your repository. For more information, see "[Viewing and updating vulnerable dependencies in your repository](/github/managing-security-vulnerabilities/viewing-and-updating-vulnerable-dependencies-in-your-repository)."
-- [Manage data usage for your private repository](/github/understanding-how-github-uses-and-protects-your-data/managing-data-use-settings-for-your-private-repository){% endif %}
-- [Define code owners for the repository](/articles/about-code-owners)
-- [Archive repositories](/articles/about-archiving-repositories){% if currentVersion == "free-pro-team@latest" %}
-- Create security advisories. For more information, see "[About {% data variables.product.prodname_security_advisories %}](/github/managing-security-vulnerabilities/about-github-security-advisories)."
-- Display a sponsor button. For more information, see "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)."{% endif %}
-
-There is only **one owner** of a repository owned by a user account; this permission cannot be shared with another user account. To transfer ownership of a repository to another user, see "[How to transfer a repository](/articles/how-to-transfer-a-repository)."
-
-#### Collaborator access on a repository owned by a user account
+### Owner access for a repository owned by a user account
+
+The repository owner has full control of the repository. In addition to the actions that any collaborator can perform, the repository owner can perform the following actions.
+
+| 동작 | More information |
+|:---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |:----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| {% if currentVersion == "free-pro-team@latest" %}Invite collaborators{% else %}Add collaborators{% endif %} | |
+| "[Inviting collaborators to a personal repository](/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository)" | |
+| Change the visibility of the repository | "[Setting repository visibility](/github/administering-a-repository/setting-repository-visibility)" |{% if currentVersion == "free-pro-team@latest" %}
+| Limit interactions with the repository | "[Limiting interactions in your repository](/github/building-a-strong-community/limiting-interactions-in-your-repository)" |{% endif %}
+| Merge a pull request on a protected branch, even if there are no approving reviews | "[About protected branches](/github/administering-a-repository/about-protected-branches)" |
+| Delete the repository | "[Deleting a repository](/github/administering-a-repository/deleting-a-repository)" |
+| Manage the repository's topics | "[Classifying your repository with topics](/github/administering-a-repository/classifying-your-repository-with-topics)" |{% if currentVersion == "free-pro-team@latest" %}
+| Manage security and analysis settings for the repository | "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository)" |{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| Enable the dependency graph for a private repository | "[Exploring the dependencies of a repository](/github/visualizing-repository-data-with-graphs/exploring-the-dependencies-of-a-repository#enabling-and-disabling-the-dependency-graph-for-a-private-repository)" |{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| Delete packages | "[Deleting a package](/github/managing-packages-with-github-packages/deleting-a-package)" |{% endif %}
+| Customize the repository's social media preview | "[Customizing your repository's social media preview](/github/administering-a-repository/customizing-your-repositorys-social-media-preview)" |
+| Create a template from the repository | "[Creating a template repository](/github/creating-cloning-and-archiving-repositories/creating-a-template-repository)" |{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+| Receive | |
+| {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot_alerts %}{% else %}security alerts{% endif %} for vulnerable dependencies | "[About alerts for vulnerable dependencies](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies)" |{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| Dismiss {% data variables.product.prodname_dependabot_alerts %} in the repository | "[Viewing and updating vulnerable dependencies in your repository](/github/managing-security-vulnerabilities/viewing-and-updating-vulnerable-dependencies-in-your-repository)" |
+| Manage data use for a private repository | "[Managing data use settings for your private repository](/github/understanding-how-github-uses-and-protects-your-data/managing-data-use-settings-for-your-private-repository)"|{% endif %}
+| Define code owners for the repository | "[About code owners](/github/creating-cloning-and-archiving-repositories/about-code-owners)" |
+| Archive the repository | "[About archiving repositories](/github/creating-cloning-and-archiving-repositories/about-archiving-repositories)" |{% if currentVersion == "free-pro-team@latest" %}
+| Create security advisories | "[About {% data variables.product.prodname_security_advisories %}](/github/managing-security-vulnerabilities/about-github-security-advisories)" |
+| Display a sponsor button | "[Displaying a sponsor button in your repository](/github/administering-a-repository/displaying-a-sponsor-button-in-your-repository)" |{% endif %}
+
+### Collaborator access for a repository owned by a user account
+
+Collaborators on a personal repository can pull (read) the contents of the repository and push (write) changes to the repository.
{% note %}
@@ -48,27 +58,26 @@ There is only **one owner** of a repository owned by a user account; this permis
{% endnote %}
-Collaborators on a personal repository can:
-
-- Push to (write), pull from (read), and fork (copy) the repository
-- Create, apply, and delete labels and milestones
-- Open, close, re-open, and assign issues
-- Edit and delete comments on commits, pull requests, and issues
-- Mark an issue or pull request as a duplicate. For more information, see "[About duplicate issues and pull requests](/articles/about-duplicate-issues-and-pull-requests)."
-- Open, merge and close pull requests
-- Apply suggested changes to pull requests. For more information, see "[Incorporating feedback in your pull request](/articles/incorporating-feedback-in-your-pull-request)."
-- Send pull requests from forks of the repository{% if currentVersion == "free-pro-team@latest" %}
-- Publish, view, and install packages. For more information, see "[Publishing and managing packages](/github/managing-packages-with-github-packages/publishing-and-managing-packages)."{% endif %}
-- Create and edit Wikis
-- Create and edit releases. For more information, see "[Managing releases in a repository](/github/administering-a-repository/managing-releases-in-a-repository).
-- Remove themselves as collaborators on the repository
-- Submit a review on a pull request that will affect its mergeability
-- Act as a designated code owner for the repository. For more information, see "[About code owners](/articles/about-code-owners)."
-- Lock a conversation. For more information, see "[Locking conversations](/articles/locking-conversations)."{% if currentVersion == "free-pro-team@latest" %}
-- Report abusive content to {% data variables.contact.contact_support %}. For more information, see "[Reporting abuse or spam](/articles/reporting-abuse-or-spam)."{% endif %}
-- Transfer an issue to a different repository. For more information, see "[Transferring an issue to another repository](/articles/transferring-an-issue-to-another-repository)."
+Collaborators can also perform the following actions.
+
+| 동작 | More information |
+|:----------------------------------------------------------------------------------------- |:------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| Fork the repository | "[About forks](/github/collaborating-with-issues-and-pull-requests/about-forks)" |
+| Create, edit, and delete comments on commits, pull requests, and issues in the repository | - "[About issues](/github/managing-your-work-on-github/about-issues)"
- "[Commenting on a pull request](/github/collaborating-with-issues-and-pull-requests/commenting-on-a-pull-request)"
- "[Managing disruptive comments](/github/building-a-strong-community/managing-disruptive-comments)"
|
+| Create, assign, close, and re-open issues in the repository | "[Managing your work with issues](/github/managing-your-work-on-github/managing-your-work-with-issues)" |
+| Manage labels for issues and pull requests in the repository | "[Labeling issues and pull requests](/github/managing-your-work-on-github/labeling-issues-and-pull-requests)" |
+| Manage milestones for issues and pull requests in the repository | "[Creating and editing milestones for issues and pull requests](/github/managing-your-work-on-github/creating-and-editing-milestones-for-issues-and-pull-requests)" |
+| Mark an issue or pull request in the repository as a duplicate | "[About duplicate issues and pull requests](/github/managing-your-work-on-github/about-duplicate-issues-and-pull-requests)" |
+| Create, merge, and close pull requests in the repository | "[Proposing changes to your work with pull requests](/github/collaborating-with-issues-and-pull-requests/proposing-changes-to-your-work-with-pull-requests)" |
+| Apply suggested changes to pull requests in the repository | "[Incorporating feedback in your pull request](/github/collaborating-with-issues-and-pull-requests/incorporating-feedback-in-your-pull-request)" |
+| Create a pull request from a fork of the repository | "[Creating a pull request from a fork](/github/collaborating-with-issues-and-pull-requests/creating-a-pull-request-from-a-fork)" |
+| Submit a review on a pull request that affects the mergeability of the pull request | "[Reviewing proposed changes in a pull request](/github/collaborating-with-issues-and-pull-requests/reviewing-proposed-changes-in-a-pull-request)" |
+| Create and edit a wiki for the repository | "[About wikis](/github/building-a-strong-community/about-wikis)" |
+| Create and edit releases for the repository | "[Managing releases in a repository](/github/administering-a-repository/managing-releases-in-a-repository)" |
+| Act as a code owner for the repository | "[About code owners](/articles/about-code-owners)" |{% if currentVersion == "free-pro-team@latest" %}
+| Publish, view, or install packages | "[Publishing and managing packages](/github/managing-packages-with-github-packages/publishing-and-managing-packages)" |{% endif %}
+| Remove themselves as collaborators on the repository | "[Removing yourself from a collaborator's repository](/github/setting-up-and-managing-your-github-user-account/removing-yourself-from-a-collaborators-repository)" |
### 더 읽을거리
-- "[Inviting collaborators to a personal repository](/articles/inviting-collaborators-to-a-personal-repository)"
- "[Repository permission levels for an organization](/articles/repository-permission-levels-for-an-organization)"
diff --git a/translations/ko-KR/content/github/site-policy/github-marketplace-terms-of-service.md b/translations/ko-KR/content/github/site-policy/github-marketplace-terms-of-service.md
index 036bc30e35a7..0d4dcedcec8d 100644
--- a/translations/ko-KR/content/github/site-policy/github-marketplace-terms-of-service.md
+++ b/translations/ko-KR/content/github/site-policy/github-marketplace-terms-of-service.md
@@ -6,11 +6,11 @@ versions:
free-pro-team: '*'
---
-Welcome to GitHub Marketplace ("Marketplace")! We're happy you're here. Please read these Terms of Service ("Marketplace Terms") carefully before accessing or using GitHub Marketplace. GitHub Marketplace is a platform that allows you to purchase developer products (for free or for a charge) that can be used with your GitHub.com account ("Developer Products"). Although sold by GitHub, Inc. ("GitHub", "we", "us"), Developer Products may be developed and maintained by either GitHub or by third-party software providers, and may require you to agree to a separate terms of service. Your use and/or purchase of Developer Products is subject to these Marketplace Terms and to the applicable fees and may also be subject to additional terms as provided by the third party licensor of that Developer Product (the "Product Provider").
+Welcome to GitHub Marketplace ("Marketplace")! We're happy you're here. Please read these Terms of Service ("Marketplace Terms") carefully before accessing or using GitHub Marketplace. GitHub Marketplace is a platform that allows you to select developer apps or actions (for free or for a charge) that can be used with your GitHub.com account ("Developer Products"). Although offered by GitHub, Inc. ("GitHub", "we", "us"), Developer Products may be developed and maintained by either GitHub or by third-party software providers. Your selection or use of Developer Products is subject to these Marketplace Terms and any applicable fees, and may require you to agree to additional terms as provided by the third party licensor of that Developer Product (the "Product Provider").
By using Marketplace, you are agreeing to be bound by these Marketplace Terms.
-Effective Date: October 11, 2017
+Effective Date: November 20, 2020
### A. GitHub.com's Terms of Service
@@ -40,11 +40,11 @@ If you would have a question, concern, or dispute regarding your billing, please
### E. Your Data and GitHub's Privacy Policy
-**Privacy.** When you purchase or subscribe to a Developer Product, GitHub must share certain Personal Information (as defined in the [GitHub Privacy Statement](/articles/github-privacy-statement/)) with the Product Provider in order to provide you with the Developer Product, regardless of your privacy settings. Depending on the requirements of the Developer Product you choose, GitHub may share as little as your user account name, ID, and primary email address or as much as access to the content in your repositories, including the ability to read and modify your private data. You will be able to view the scope of the permissions the Developer Product is requesting, and accept or deny them, when you grant it authorization via OAuth. In line with [GitHub's Privacy Statement](/articles/github-privacy-statement/), we will only provide the Product Provider with the minimum amount of information necessary for the purpose of the transaction.
+**Privacy.** When you select or use a Developer Product, GitHub may share certain Personal Information (as defined in the [GitHub Privacy Statement](/articles/github-privacy-statement/)) with the Product Provider (if any such Personal Information is received from you) in order to provide you with the Developer Product, regardless of your privacy settings. Depending on the requirements of the Developer Product you choose, GitHub may share as little as your user account name, ID, and primary email address or as much as access to the content in your repositories, including the ability to read and modify your private data. You will be able to view the scope of the permissions the Developer Product is requesting, and accept or deny them, when you grant it authorization via OAuth.
-If you cancel your Developer Product services and revoke access through your account settings, the Product Provider will no longer be able to access your account. The Product Provider is responsible for deleting your Personal Information from its systems within its defined window. Please contact the Product Provider to ensure that your account has been properly terminated.
+If you cease using the Developer Product and revoke access through your account settings, the Product Provider will no longer be able to access your account. The Product Provider is responsible for deleting your Personal Information from its systems within its defined window. Please contact the Product Provider to ensure that your account has been properly terminated.
-**Data Security Disclaimer.** When you purchase or subscribe to a Developer Product, the Developer Product security and their custodianship of your data is the responsibility of the Product Provider. You are responsible for understanding the security considerations of the purchase and use of the Developer Product for your own security, risk and compliance considerations.
+**Data Security and Privacy Disclaimer.** When you select or use a Developer Product, the security of the Developer Product and the custodianship of your data, including your Personal Information (if any), is the responsibility of the Product Provider. You are responsible for understanding the security and privacy considerations of the selection or use of the Developer Product for your own security and privacy risk and compliance considerations.
### F. Rights to Developer Products
diff --git a/translations/ko-KR/content/github/using-git/setting-your-username-in-git.md b/translations/ko-KR/content/github/using-git/setting-your-username-in-git.md
index 8352c098af7b..c958954661f5 100644
--- a/translations/ko-KR/content/github/using-git/setting-your-username-in-git.md
+++ b/translations/ko-KR/content/github/using-git/setting-your-username-in-git.md
@@ -20,13 +20,13 @@ Changing the name associated with your Git commits using `git config` will only
2. {% data reusables.user_settings.set_your_git_username %}
```shell
$ git config --global user.name "Mona Lisa"
- ```
+ ```
3. {% data reusables.user_settings.confirm_git_username_correct %}
```shell
$ git config --global user.name
> Mona Lisa
- ```
+ ```
### Setting your Git username for a single repository
@@ -37,13 +37,13 @@ Changing the name associated with your Git commits using `git config` will only
3. {% data reusables.user_settings.set_your_git_username %}
```shell
$ git config user.name "Mona Lisa"
- ```
+ ```
3. {% data reusables.user_settings.confirm_git_username_correct %}
```shell
$ git config user.name
> Mona Lisa
- ```
+ ```
### 더 읽을거리
diff --git a/translations/ko-KR/content/github/using-git/which-remote-url-should-i-use.md b/translations/ko-KR/content/github/using-git/which-remote-url-should-i-use.md
index 6ebdb3c5fd98..961edb4c57da 100644
--- a/translations/ko-KR/content/github/using-git/which-remote-url-should-i-use.md
+++ b/translations/ko-KR/content/github/using-git/which-remote-url-should-i-use.md
@@ -3,7 +3,7 @@ title: Which remote URL should I use?
redirect_from:
- /articles/which-url-should-i-use/
- /articles/which-remote-url-should-i-use
-intro: 'There are several ways to clone repositories available on {% data variables.product.prodname_dotcom %}.'
+intro: 'There are several ways to clone repositories available on {% data variables.product.product_location %}.'
versions:
free-pro-team: '*'
enterprise-server: '*'
@@ -16,7 +16,7 @@ For information on setting or changing your remote URL, see "[Changing a remote'
### Cloning with HTTPS URLs
-The `https://` clone URLs are available on all repositories, public and private. These URLs work even if you are behind a firewall or proxy.
+The `https://` clone URLs are available on all repositories, regardless of visibility. `https://` clone URLs work even if you are behind a firewall or proxy.
When you `git clone`, `git fetch`, `git pull`, or `git push` to a remote repository using HTTPS URLs on the command line, Git will ask for your {% data variables.product.product_name %} username and password. {% data reusables.user_settings.password-authentication-deprecation %}
diff --git a/translations/ko-KR/content/github/writing-on-github/creating-gists.md b/translations/ko-KR/content/github/writing-on-github/creating-gists.md
index 0f68b324b6ea..d5655b46bb08 100644
--- a/translations/ko-KR/content/github/writing-on-github/creating-gists.md
+++ b/translations/ko-KR/content/github/writing-on-github/creating-gists.md
@@ -33,8 +33,12 @@ You'll receive a notification when:
- Someone mentions you in a gist.
- You subscribe to a gist, by clicking **Subscribe** at the top any gist.
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
You can pin gists to your profile so other people can see them easily. For more information, see "[Pinning items to your profile](/articles/pinning-items-to-your-profile)."
+{% endif %}
+
You can discover gists others have created by going to the {% data variables.gists.gist_homepage %} and clicking **All Gists**. This will take you to a page of all gists sorted and displayed by time of creation or update. You can also search gists by language with {% data variables.gists.gist_search_url %}. Gist search uses the same search syntax as [code search](/articles/searching-code).
Since gists are Git repositories, you can view their full commit history, complete with diffs. You can also fork or clone gists. For more information, see ["Forking and cloning gists"](/articles/forking-and-cloning-gists).
diff --git a/translations/ko-KR/content/packages/managing-container-images-with-github-container-registry/deleting-a-container-image.md b/translations/ko-KR/content/packages/managing-container-images-with-github-container-registry/deleting-a-container-image.md
index ddf6eae62764..3fd9ac99bd21 100644
--- a/translations/ko-KR/content/packages/managing-container-images-with-github-container-registry/deleting-a-container-image.md
+++ b/translations/ko-KR/content/packages/managing-container-images-with-github-container-registry/deleting-a-container-image.md
@@ -16,10 +16,6 @@ To delete a container image, you must have admin permissions to the container im
When deleting public packages, be aware that you may break projects that depend on your package.
-### Reserved package versions and names
-
-{% data reusables.package_registry.package-immutability %}
-
### Deleting a version of a user-owned container image on {% data variables.product.prodname_dotcom %}
{% data reusables.package_registry.package-settings-from-user-level %}
diff --git a/translations/ko-KR/content/packages/publishing-and-managing-packages/deleting-a-package.md b/translations/ko-KR/content/packages/publishing-and-managing-packages/deleting-a-package.md
index dceaa32d12c9..7ce43daf8b0c 100644
--- a/translations/ko-KR/content/packages/publishing-and-managing-packages/deleting-a-package.md
+++ b/translations/ko-KR/content/packages/publishing-and-managing-packages/deleting-a-package.md
@@ -35,10 +35,6 @@ At this time, {% data variables.product.prodname_registry %} on {% data variable
{% endif %}
-### Reserved package versions and names
-
-{% data reusables.package_registry.package-immutability %}
-
### Deleting a version of a private package on {% data variables.product.product_name %}
To delete a private package version, you must have admin permissions in the repository.
diff --git a/translations/ko-KR/content/packages/publishing-and-managing-packages/publishing-a-package.md b/translations/ko-KR/content/packages/publishing-and-managing-packages/publishing-a-package.md
index d016cc2ba7c9..406c897528f5 100644
--- a/translations/ko-KR/content/packages/publishing-and-managing-packages/publishing-a-package.md
+++ b/translations/ko-KR/content/packages/publishing-and-managing-packages/publishing-a-package.md
@@ -18,8 +18,6 @@ You can help people understand and use your package by providing a description a
{% data reusables.package_registry.public-or-private-packages %} A repository can contain more than one package. To prevent confusion, make sure the README and description clearly provide information about each package.
-{% data reusables.package_registry.package-immutability %}
-
{% if currentVersion == "free-pro-team@latest" %}
If a new version of a package fixes a security vulnerability, you should publish a security advisory in your repository.
{% data variables.product.prodname_dotcom %} reviews each published security advisory and may use it to send {% data variables.product.prodname_dependabot_alerts %} to affected repositories. For more information, see "[About GitHub Security Advisories](/github/managing-security-vulnerabilities/about-github-security-advisories)."
diff --git a/translations/ko-KR/content/packages/using-github-packages-with-your-projects-ecosystem/configuring-npm-for-use-with-github-packages.md b/translations/ko-KR/content/packages/using-github-packages-with-your-projects-ecosystem/configuring-npm-for-use-with-github-packages.md
index 65153a6bd901..5353abe883a0 100644
--- a/translations/ko-KR/content/packages/using-github-packages-with-your-projects-ecosystem/configuring-npm-for-use-with-github-packages.md
+++ b/translations/ko-KR/content/packages/using-github-packages-with-your-projects-ecosystem/configuring-npm-for-use-with-github-packages.md
@@ -45,12 +45,15 @@ If your instance has subdomain isolation disabled:
To authenticate by logging in to npm, use the `npm login` command, replacing *USERNAME* with your {% data variables.product.prodname_dotcom %} username, *TOKEN* with your personal access token, and *PUBLIC-EMAIL-ADDRESS* with your email address.
+If {% data variables.product.prodname_registry %} is not your default package registry for using npm and you want to use the `npm audit` command, we recommend you use the `--scope` flag with the owner of the package when you authenticate to {% data variables.product.prodname_registry %}.
+
{% if enterpriseServerVersions contains currentVersion %}
If your instance has subdomain isolation enabled:
{% endif %}
```shell
-$ npm login --registry=https://{% if currentVersion == "free-pro-team@latest" %}npm.pkg.github.com{% else %}npm.HOSTNAME/{% endif %}
+$ npm login --scope=@OWNER --registry=https://{% if currentVersion == "free-pro-team@latest" %}npm.pkg.github.com{% else %}npm.HOSTNAME/{% endif %}
+
> Username: USERNAME
> Password: TOKEN
> Email: PUBLIC-EMAIL-ADDRESS
@@ -60,7 +63,7 @@ $ npm login --registry=https://{% if currentVersion == "free-pro-team@latest" %}
If your instance has subdomain isolation disabled:
```shell
-$ npm login --registry=https://HOSTNAME/_registry/npm/
+$ npm login --scope=@OWNER --registry=https://HOSTNAME/_registry/npm/
> Username: USERNAME
> Password: TOKEN
> Email: PUBLIC-EMAIL-ADDRESS
diff --git a/translations/ko-KR/data/reusables/enterprise-accounts/enterprise-accounts-billing.md b/translations/ko-KR/data/reusables/enterprise-accounts/enterprise-accounts-billing.md
index cd4984777d60..1644a4007eaa 100644
--- a/translations/ko-KR/data/reusables/enterprise-accounts/enterprise-accounts-billing.md
+++ b/translations/ko-KR/data/reusables/enterprise-accounts/enterprise-accounts-billing.md
@@ -1 +1 @@
-Enterprise accounts are currently available to {% data variables.product.prodname_ghe_cloud %} and {% data variables.product.prodname_ghe_server %} customers paying by invoice. Billing for all of the organizations and {% data variables.product.prodname_ghe_server %} instances connected to your enterprise account is aggregated into a single bill. For more information about managing your {% data variables.product.prodname_ghe_cloud %} subscription, see "[Viewing the subscription and usage for your enterprise account](/articles/viewing-the-subscription-and-usage-for-your-enterprise-account)." For more information about managing your {% data variables.product.prodname_ghe_server %} billing settings, see "[Managing billing for your enterprise](/admin/overview/managing-billing-for-your-enterprise)."
+Enterprise accounts are currently available to {% data variables.product.prodname_ghe_cloud %} and {% data variables.product.prodname_ghe_server %} customers paying by invoice. Billing for all of the organizations and {% data variables.product.prodname_ghe_server %} instances connected to your enterprise account is aggregated into a single bill.
diff --git a/translations/ko-KR/data/reusables/enterprise_installation/hardware-considerations-all-platforms.md b/translations/ko-KR/data/reusables/enterprise_installation/hardware-considerations-all-platforms.md
index b3e2e9022c5a..1ef7257106f6 100644
--- a/translations/ko-KR/data/reusables/enterprise_installation/hardware-considerations-all-platforms.md
+++ b/translations/ko-KR/data/reusables/enterprise_installation/hardware-considerations-all-platforms.md
@@ -1,4 +1,5 @@
- [Minimum requirements](#minimum-requirements)
+- [Beta features in {% data variables.product.prodname_ghe_server %} 2.22](#beta-features-in-github-enterprise-server-222)
- [Storage](#storage)
- [CPU and memory](#cpu-and-memory)
@@ -6,23 +7,18 @@
We recommend different hardware configurations depending on the number of user licenses for {% data variables.product.product_location %}. If you provision more resources than the minimum requirements, your instance will perform and scale better.
-{% data reusables.enterprise_installation.hardware-rec-table %} For more information about adjusting resources for an existing instance, see "[Increasing storage capacity](/enterprise/admin/installation/increasing-storage-capacity)" and "[Increasing CPU or memory resources](/enterprise/admin/installation/increasing-cpu-or-memory-resources)."
-
-{% if currentVersion == "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
-
-If you enable the beta for {% data variables.product.prodname_actions %} on your instance, we recommend planning for additional capacity.
+{% data reusables.enterprise_installation.hardware-rec-table %}{% if currentVersion == "enterprise-server@2.22" or currentVersion == "github-ae@latest" %} If you enable the beta for {% data variables.product.prodname_actions %}, review the following requirements and recommendations.
- You must configure at least one runner for {% data variables.product.prodname_actions %} workflows. For more information, see "[About self-hosted runners](/actions/hosting-your-own-runners/about-self-hosted-runners)."
- You must configure external blob storage. For more information, see "[Enabling {% data variables.product.prodname_actions %} and configuring storage](/enterprise/admin/github-actions/enabling-github-actions-and-configuring-storage)."
-
-The additional CPU and memory resources you need to provision for your instance depend on the number of workflows your users run concurrently, and the overall levels of activity for users, automations, and integrations.
-
-| Maximum jobs per minute | vCPUs | Memory |
-|:----------------------- | -----:| -------:|
-| Light testing | 4 | 30.5 GB |
-| 25 | 8 | 61 GB |
-| 35 | 16 | 122 GB |
-| 100 | 32 | 244 GB |
+- You may need to configure additional CPU and memory resources. The additional resources you need to provision for {% data variables.product.prodname_actions %} depend on the number of workflows your users run concurrently, and the overall levels of activity for users, automations, and integrations.
+
+ | Maximum jobs per minute | Additional vCPUs | Additional memory |
+ |:----------------------- | ----------------:| -----------------:|
+ | Light testing | 4 | 30.5 GB |
+ | 25 | 8 | 61 GB |
+ | 35 | 16 | 122 GB |
+ | 100 | 32 | 244 GB |
{% endif %}
diff --git a/translations/ko-KR/data/reusables/enterprise_installation/hardware-rec-table.md b/translations/ko-KR/data/reusables/enterprise_installation/hardware-rec-table.md
index 4de4ad712004..97997e7d4f3a 100644
--- a/translations/ko-KR/data/reusables/enterprise_installation/hardware-rec-table.md
+++ b/translations/ko-KR/data/reusables/enterprise_installation/hardware-rec-table.md
@@ -1,6 +1,12 @@
{% if currentVersion == "enterprise-server@2.22" %}
-Minimum requirements for an instance with beta features enabled are **bold** in the following table. For more information, see "[Beta features in {% data variables.product.prodname_ghe_server %} 2.22](#beta-features-in-github-enterprise-server-222)." |{% endif %}
+{% note %}
+
+**Note**: If you enable beta features for {% data variables.product.prodname_ghe_server %} 2.22, your instance requires additional hardware resources. Minimum requirements for an instance with beta features enabled are **bold** in the following table. For more information about the features in beta, see "[Beta features in {% data variables.product.prodname_ghe_server %} 2.22](#beta-features-in-github-enterprise-server-222)."
+
+{% endnote %}
+|
+{% endif %}
| User licenses | vCPUs | Memory | Attached storage | Root storage |
|:------------------------------ | --------------------------------------------------------------------------------------------------------------------------------------:| ----------------------------------------------------------------------------------------------------------------------------------------------:| ----------------------------------------------------------------------------------------------------------------------------------------------:| ------------:|
| Trial, demo, or 10 light users | 2{% if currentVersion == "enterprise-server@2.22" %}
or [**4**](#beta-features-in-github-enterprise-server-222){% endif %} | 16 GB{% if currentVersion == "enterprise-server@2.22" %}
or [**32 GB**](#beta-features-in-github-enterprise-server-222){% endif %} | 100 GB{% if currentVersion == "enterprise-server@2.22" %}
or [**150 GB**](#beta-features-in-github-enterprise-server-222){% endif %} | 200 GB |
@@ -9,8 +15,14 @@ Minimum requirements for an instance with beta features enabled are **bold** in
| 5,000 to 8000 | 12{% if currentVersion == "enterprise-server@2.22" %}
or [**16**](#beta-features-in-github-enterprise-server-222){% endif %} | 96 GB | 750 GB | 200 GB |
| 8,000 to 10,000+ | 16{% if currentVersion == "enterprise-server@2.22" %}
or [**20**](#beta-features-in-github-enterprise-server-222){% endif %} | 128 GB{% if currentVersion == "enterprise-server@2.22" %}
or [**160 GB**](#beta-features-in-github-enterprise-server-222){% endif %} | 1000 GB | 200 GB |
+For more information about adjusting resources for an existing instance, see "[Increasing storage capacity](/enterprise/admin/installation/increasing-storage-capacity)" and "[Increasing CPU or memory resources](/enterprise/admin/installation/increasing-cpu-or-memory-resources)."
+
{% if currentVersion == "enterprise-server@2.22" %}
#### Beta features in {% data variables.product.prodname_ghe_server %} 2.22
-If you enable beta features in {% data variables.product.prodname_ghe_server %} 2.22, your instance requires additional hardware resources. For more information about the beta features, see the [release notes for the 2.22 series](https://enterprise.github.com/releases/series/2.22) on the {% data variables.product.prodname_enterprise %} website.{% endif %}
+You can sign up for beta features available in {% data variables.product.prodname_ghe_server %} 2.22 such as {% data variables.product.prodname_actions %}, {% data variables.product.prodname_registry %}, and {% data variables.product.prodname_code_scanning %}. For more information, see the [release notes for the 2.22 series](https://enterprise.github.com/releases/series/2.22#release-2.22.0) on the {% data variables.product.prodname_enterprise %} website.
+
+If you enable beta features for {% data variables.product.prodname_ghe_server %} 2.22, your instance requires additional hardware resources. For more information, see "[Minimum requirements](#minimum-requirements)".
+
+{% endif %}
diff --git a/translations/ko-KR/data/reusables/enterprise_installation/image-urls-viewable-warning.md b/translations/ko-KR/data/reusables/enterprise_installation/image-urls-viewable-warning.md
index 19b842266e07..2a26ad6d64f7 100644
--- a/translations/ko-KR/data/reusables/enterprise_installation/image-urls-viewable-warning.md
+++ b/translations/ko-KR/data/reusables/enterprise_installation/image-urls-viewable-warning.md
@@ -1,5 +1,5 @@
{% warning %}
-**Warning:** If you add an image attachment to a pull request or issue comment, anyone can view the anonymized image URL without authentication, even if the pull request is in a private repository, or if private mode is enabled. To keep sensitive images private, serve them from a private network or server that requires authentication.
+**Warning:** If you add an image attachment to a pull request or issue comment, anyone can view the anonymized image URL without authentication{% if enterpriseServerVersions contains currentVersion %}, even if the pull request is in a private repository, or if private mode is enabled. To prevent unauthorized access to the images, ensure that you restrict network access to the systems that serve the images, including {% data variables.product.product_location %}{% endif %}.{% if currentVersion == "github-ae@latest" %} To prevent unauthorized access to image URLs on {% data variables.product.product_name %}, consider restricting network traffic to your enterprise. For more information, see "[Restricting network traffic to your enterprise](/admin/configuration/restricting-network-traffic-to-your-enterprise)."{% endif %}
{% endwarning %}
diff --git a/translations/ko-KR/data/reusables/gated-features/pages.md b/translations/ko-KR/data/reusables/gated-features/pages.md
index e24925cc9877..31caf392d2d3 100644
--- a/translations/ko-KR/data/reusables/gated-features/pages.md
+++ b/translations/ko-KR/data/reusables/gated-features/pages.md
@@ -1 +1 @@
-{% data variables.product.prodname_pages %} is available in public repositories with {% data variables.product.prodname_free_user %} and {% data variables.product.prodname_free_team %} for organizations, and in public and private repositories with {% data variables.product.prodname_pro %}, {% data variables.product.prodname_team %}, {% data variables.product.prodname_ghe_cloud %},{% if currentVersion == "github-ae@latest" %} {% data variables.product.prodname_ghe_managed %},{% endif %} and {% data variables.product.prodname_ghe_server %}. {% if currentVersion == "free-pro-team@latest" %}{% data reusables.gated-features.more-info %}{% endif %}
+{% if currentVersion == "github-ae@latest" %}{% data variables.product.prodname_pages %} is available in internal and private repositories with {% data variables.product.prodname_ghe_managed %}. {% endif %}{% data variables.product.prodname_pages %} is available in public repositories with {% data variables.product.prodname_free_user %} and {% data variables.product.prodname_free_team %} for organizations, and in public and private repositories with {% data variables.product.prodname_pro %}, {% data variables.product.prodname_team %}, {% data variables.product.prodname_ghe_cloud %}, and {% data variables.product.prodname_ghe_server %}. {% if currentVersion == "free-pro-team@latest" %}{% data reusables.gated-features.more-info %}{% endif %}
diff --git a/translations/ko-KR/data/reusables/gated-features/wikis.md b/translations/ko-KR/data/reusables/gated-features/wikis.md
index 61f560896e02..d7611dc3638c 100644
--- a/translations/ko-KR/data/reusables/gated-features/wikis.md
+++ b/translations/ko-KR/data/reusables/gated-features/wikis.md
@@ -1 +1 @@
-Wikis are available in public repositories with {% data variables.product.prodname_free_user %} and {% data variables.product.prodname_free_team %} for organizations, and in public and private repositories with {% data variables.product.prodname_pro %}, {% data variables.product.prodname_team %}, {% data variables.product.prodname_ghe_cloud %},{% if currentVersion == "github-ae@latest" %} {% data variables.product.prodname_ghe_managed %},{% endif %} and {% data variables.product.prodname_ghe_server %}. {% if currentVersion == "free-pro-team@latest" %}{% data reusables.gated-features.more-info %}{% endif %}
+{% if currentVersion == "github-ae@latest" %}Wikis are available in internal and private repositories with {% data variables.product.prodname_ghe_managed %}.{% endif %} Wikis are available in public repositories with {% data variables.product.prodname_free_user %} and {% data variables.product.prodname_free_team %} for organizations, and in public and private repositories with {% data variables.product.prodname_pro %}, {% data variables.product.prodname_team %}, {% data variables.product.prodname_ghe_cloud %} and {% data variables.product.prodname_ghe_server %}. {% if currentVersion == "free-pro-team@latest" %}{% data reusables.gated-features.more-info %}{% endif %}
diff --git a/translations/ko-KR/data/reusables/github-actions/self-hosted-runner-configure-runner-group-access.md b/translations/ko-KR/data/reusables/github-actions/self-hosted-runner-configure-runner-group-access.md
index 59d3343c244e..9ac93498fa1e 100644
--- a/translations/ko-KR/data/reusables/github-actions/self-hosted-runner-configure-runner-group-access.md
+++ b/translations/ko-KR/data/reusables/github-actions/self-hosted-runner-configure-runner-group-access.md
@@ -1,2 +1,12 @@
-1. In the **Self-hosted runners** section of the settings page, click {% octicon "kebab-horizontal" aria-label="The horizontal kebab icon" %} next to the runner group you'd like to configure, then click **Edit name and [organization|repository] access**. 
-1. Select a new policy from the dropdown list, or modify the runner group name.
+1. In the **Self-hosted runners** section of the settings page, click {% octicon "kebab-horizontal" aria-label="The horizontal kebab icon" %} next to the runner group you'd like to configure, then click **Edit name and [organization|repository] access**.
+ 
+1. Modify your policy options, or change the runner group name.
+
+ {% warning %}
+
+ **Warning**
+ {% indented_data_reference site.data.reusables.github-actions.self-hosted-runner-security spaces=3 %}
+ For more information, see "[About self-hosted runners](/actions/hosting-your-own-runners/about-self-hosted-runners#self-hosted-runner-security-with-public-repositories)."
+
+ {% endwarning %}
+
diff --git a/translations/ko-KR/data/reusables/notifications-v2/custom-notifications-beta.md b/translations/ko-KR/data/reusables/notifications-v2/custom-notifications-beta.md
new file mode 100644
index 000000000000..dbcee80cfe1d
--- /dev/null
+++ b/translations/ko-KR/data/reusables/notifications-v2/custom-notifications-beta.md
@@ -0,0 +1,9 @@
+{% if currentVersion == "free-pro-team@latest" %}
+
+{% note %}
+
+**Note:** Custom notifications are currently in beta and subject to change.
+
+{% endnote %}
+
+{% endif %}
diff --git a/translations/ko-KR/data/reusables/notifications/outbound_email_tip.md b/translations/ko-KR/data/reusables/notifications/outbound_email_tip.md
index 6e261ac61517..1cd91b0775f1 100644
--- a/translations/ko-KR/data/reusables/notifications/outbound_email_tip.md
+++ b/translations/ko-KR/data/reusables/notifications/outbound_email_tip.md
@@ -1,7 +1,9 @@
-{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
- {% tip %}
+{% if enterpriseServerVersions contains currentVersion %}
- You'll only receive email notifications if outbound email support is enabled on {% data variables.product.product_location %}. For more information, contact your site administrator.
+{% note %}
+
+**Note**: You'll only receive email notifications if outbound email support is enabled on {% data variables.product.product_location %}. For more information, contact your site administrator.
+
+{% endnote %}
- {% endtip %}
{% endif %}
diff --git a/translations/ko-KR/data/reusables/notifications/shared_state.md b/translations/ko-KR/data/reusables/notifications/shared_state.md
index f9a4d8b42757..d6b53f667575 100644
--- a/translations/ko-KR/data/reusables/notifications/shared_state.md
+++ b/translations/ko-KR/data/reusables/notifications/shared_state.md
@@ -1,5 +1,5 @@
{% tip %}
-**Tip:** If you receive both web and email notifications, you can automatically sync the read or unread status of the notification so that web notifications are automatically marked as read once you've read the corresponding email notification. To enable this sync, your email client must be able to view images from {% if currentVersion == "free-pro-team@latest" %}'`notifications@github.com`'{% else %}'the no-reply email address configured by your site administrator'{% endif %}.
+**Tip:** If you receive both web and email notifications, you can automatically sync the read or unread status of the notification so that web notifications are automatically marked as read once you've read the corresponding email notification. To enable this sync, your email client must be able to view images from {% if currentVersion == "free-pro-team@latest" %}`notifications@github.com`{% else %}the `no-reply` email address {% if currentVersion == "github-ae@latest" %}for your {% data variables.product.product_name %} hostname{% elsif enterpriseServerVersions contains currentVersion %}for {% data variables.product.product_location %}, which your site administrator configures{% endif %}{% endif %}.
{% endtip %}
diff --git a/translations/ko-KR/data/reusables/profile/profile-readme.md b/translations/ko-KR/data/reusables/profile/profile-readme.md
index a19a3d4a30d3..7350ed57bd30 100644
--- a/translations/ko-KR/data/reusables/profile/profile-readme.md
+++ b/translations/ko-KR/data/reusables/profile/profile-readme.md
@@ -1 +1 @@
-If you add a README file to the root of a public repository with the same name as your username, that README will automatically appear on your profile page. You can edit your profile README with GitHub Flavored Markdown to create a personalized section on your profile.
+If you add a README file to the root of a public repository with the same name as your username, that README will automatically appear on your profile page. You can edit your profile README with {% data variables.product.company_short %} Flavored Markdown to create a personalized section on your profile. For more information, see "[Managing your profile README](/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme)."
diff --git a/translations/ko-KR/data/reusables/repositories/administrators-can-disable-issues.md b/translations/ko-KR/data/reusables/repositories/administrators-can-disable-issues.md
new file mode 100644
index 000000000000..19c435397a28
--- /dev/null
+++ b/translations/ko-KR/data/reusables/repositories/administrators-can-disable-issues.md
@@ -0,0 +1 @@
+Repository administrators can disable issues for a repository. For more information, see "[Disabling issues](/github/managing-your-work-on-github/disabling-issues)."
diff --git a/translations/ko-KR/data/reusables/repositories/desktop-fork.md b/translations/ko-KR/data/reusables/repositories/desktop-fork.md
index cf7bb2d54690..c7d52b1a88b1 100644
--- a/translations/ko-KR/data/reusables/repositories/desktop-fork.md
+++ b/translations/ko-KR/data/reusables/repositories/desktop-fork.md
@@ -1 +1 @@
-You can use {% data variables.product.prodname_desktop %} to fork a repository. For more information, see “[Cloning and forking repositories from {% data variables.product.prodname_desktop %}](/desktop/contributing-to-projects/cloning-and-forking-repositories-from-github-desktop)."
+You can use {% data variables.product.prodname_desktop %} to fork a repository. For more information, see "[Cloning and forking repositories from {% data variables.product.prodname_desktop %}](/desktop/contributing-to-projects/cloning-and-forking-repositories-from-github-desktop)."
diff --git a/translations/ko-KR/data/reusables/repositories/you-can-fork.md b/translations/ko-KR/data/reusables/repositories/you-can-fork.md
index 2d290ce6cde4..e72953648ddb 100644
--- a/translations/ko-KR/data/reusables/repositories/you-can-fork.md
+++ b/translations/ko-KR/data/reusables/repositories/you-can-fork.md
@@ -1,3 +1,7 @@
-You can fork any public repository to your user account or any organization where you have repository creation permissions. For more information, see "[Permission levels for an organization](/articles/permission-levels-for-an-organization)."
+{% if currentVersion == "github-ae@latest" %}If the policies for your enterprise permit forking internal and private repositories, you{% else %}You{% endif %} can fork a repository to your user account or any organization where you have repository creation permissions. For more information, see "[Permission levels for an organization](/articles/permission-levels-for-an-organization)."
-You can fork any private repository you can access to your user account and any organization on {% data variables.product.prodname_team %} or {% data variables.product.prodname_enterprise %} where you have repository creation permissions. You cannot fork a private repository to an organization using {% data variables.product.prodname_free_team %}.{% if currentVersion == "free-pro-team@latest" %} For more information, see "[GitHub's products](/articles/githubs-products)."{% endif %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+If you have access to a private repository and the owner permits forking, you can fork the repository to your user account or any organization on {% if currentVersion == "free-pro-team@latest"%}{% data variables.product.prodname_team %}{% else %}{% data variables.product.product_location %}{% endif %} where you have repository creation permissions. {% if currentVersion == "free-pro-team@latest" %}You cannot fork a private repository to an organization using {% data variables.product.prodname_free_team %}. For more information, see "[GitHub's products](/articles/githubs-products)."{% endif %}
+
+{% endif %}
\ No newline at end of file
diff --git a/translations/ko-KR/data/reusables/search/required_login.md b/translations/ko-KR/data/reusables/search/required_login.md
index 813810508ced..6d8598a94a38 100644
--- a/translations/ko-KR/data/reusables/search/required_login.md
+++ b/translations/ko-KR/data/reusables/search/required_login.md
@@ -1 +1 @@
-You must be signed in to search for code across all public repositories.
+You must be signed into a user account on {% data variables.product.product_name %} to search for code across all public repositories.
diff --git a/translations/ko-KR/data/reusables/search/syntax_tips.md b/translations/ko-KR/data/reusables/search/syntax_tips.md
index 9470b7f01a9d..363da832722a 100644
--- a/translations/ko-KR/data/reusables/search/syntax_tips.md
+++ b/translations/ko-KR/data/reusables/search/syntax_tips.md
@@ -1,7 +1,7 @@
{% tip %}
**Tips:**{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
- - This article contains example searches on the {% data variables.product.prodname_dotcom %}.com website, but you can use the same search filters on {% data variables.product.product_location %}.{% endif %}
+ - This article contains links to example searches on the {% data variables.product.prodname_dotcom_the_website %} website, but you can use the same search filters with {% data variables.product.product_name %}. In the linked example searches, replace `github.com` with the hostname for {% data variables.product.product_location %}.{% endif %}
- For a list of search syntaxes that you can add to any search qualifier to further improve your results, see "[Understanding the search syntax](/articles/understanding-the-search-syntax)".
- Use quotations around multi-word search terms. For example, if you want to search for issues with the label "In progress," you'd search for `label:"in progress"`. Search is not case sensitive.
diff --git a/translations/ko-KR/data/reusables/search/you-can-search-globally.md b/translations/ko-KR/data/reusables/search/you-can-search-globally.md
new file mode 100644
index 000000000000..dd690bc80fe1
--- /dev/null
+++ b/translations/ko-KR/data/reusables/search/you-can-search-globally.md
@@ -0,0 +1 @@
+You can search globally across all of {% data variables.product.product_name %}, or scope your search to a particular repository or organization.
diff --git a/translations/ko-KR/data/reusables/ssh/add-ssh-key-to-ssh-agent-commandline.md b/translations/ko-KR/data/reusables/ssh/add-ssh-key-to-ssh-agent-commandline.md
index af4760bd1725..415f86736de3 100644
--- a/translations/ko-KR/data/reusables/ssh/add-ssh-key-to-ssh-agent-commandline.md
+++ b/translations/ko-KR/data/reusables/ssh/add-ssh-key-to-ssh-agent-commandline.md
@@ -1,3 +1,3 @@
```shell
-$ ssh-add ~/.ssh/id_rsa
+$ ssh-add ~/.ssh/id_ed25519
```
diff --git a/translations/ko-KR/data/reusables/ssh/add-ssh-key-to-ssh-agent.md b/translations/ko-KR/data/reusables/ssh/add-ssh-key-to-ssh-agent.md
index 6a2f98182365..fb1ca5ec3428 100644
--- a/translations/ko-KR/data/reusables/ssh/add-ssh-key-to-ssh-agent.md
+++ b/translations/ko-KR/data/reusables/ssh/add-ssh-key-to-ssh-agent.md
@@ -1 +1 @@
-If you created your key with a different name, or if you are adding an existing key that has a different name, replace *id_rsa* in the command with the name of your private key file.
+If you created your key with a different name, or if you are adding an existing key that has a different name, replace *id_ed25519* in the command with the name of your private key file.
diff --git a/translations/ko-KR/data/variables/action_code_examples.yml b/translations/ko-KR/data/variables/action_code_examples.yml
index c35362989200..d68a26f48ae1 100644
--- a/translations/ko-KR/data/variables/action_code_examples.yml
+++ b/translations/ko-KR/data/variables/action_code_examples.yml
@@ -6,7 +6,7 @@
href: actions/starter-workflows
tags:
- official
- - workflows
+ - 워크플로
-
title: Example services
description: Example workflows using service containers
@@ -64,7 +64,7 @@
- publishing
-
title: GitHub Project Automation+
- description: Automate GitHub Project cards with any webhook event.
+ description: Automate GitHub Project cards with any webhook event
languages: JavaScript
href: alex-page/github-project-automation-plus
tags:
@@ -131,8 +131,7 @@
- publishing
-
title: Label your Pull Requests auto-magically (using committed files)
- description: >-
- Github action to label your pull requests auto-magically (using committed files)
+ description: Github action to label your pull requests auto-magically (using committed files)
languages: 'TypeScript, Dockerfile, JavaScript'
href: Decathlon/pull-request-labeler-action
tags:
@@ -147,3 +146,191 @@
tags:
- 끌어오기 요청
- labels
+-
+ title: Get a list of file changes with a PR/Push
+ description: This action gives you will get outputs of the files that have changed in your repository
+ languages: 'TypeScript, Shell, JavaScript'
+ href: trilom/file-changes-action
+ tags:
+ - 워크플로
+ - 리포지토리
+-
+ title: Private actions in any workflow
+ description: Allows private GitHub Actions to be easily reused
+ languages: 'TypeScript, JavaScript, Shell'
+ href: InVisionApp/private-action-loader
+ tags:
+ - 워크플로
+ - tools
+-
+ title: Label your issues using the issue's contents
+ description: A GitHub Action to automatically tag issues with labels and assignees
+ languages: 'JavaScript, TypeScript'
+ href: damccorm/tag-ur-it
+ tags:
+ - 워크플로
+ - tools
+ - labels
+ - issues
+-
+ title: Rollback a GitHub Release
+ description: A GitHub Action to rollback or delete a release
+ languages: 'JavaScript'
+ href: author/action-rollback
+ tags:
+ - 워크플로
+ - 버전 출시
+-
+ title: Lock closed issues and Pull Requests
+ description: GitHub Action that locks closed issues and pull requests after a period of inactivity
+ languages: 'JavaScript'
+ href: dessant/lock-threads
+ tags:
+ - issues
+ - pull requests
+ - 워크플로
+-
+ title: Get Commit Difference Count Between Two Branches
+ description: This GitHub Action compares two branches and gives you the commit count between them
+ languages: 'JavaScript, Shell'
+ href: jessicalostinspace/commit-difference-action
+ tags:
+ - 커밋
+ - 비교(diff)
+ - 워크플로
+-
+ title: Generate Release Notes Based on Git References
+ description: GitHub Action to generate changelogs, and release notes
+ languages: 'JavaScript, Shell'
+ href: metcalfc/changelog-generator
+ tags:
+ - cicd
+ - release-notes
+ - 워크플로
+ - changelog
+-
+ title: Enforce Policies on GitHub Repositories and Commits
+ description: Policy enforcement for your pipelines
+ languages: 'Go, Makefile, Dockerfile, Shell'
+ href: talos-systems/conform
+ tags:
+ - docker
+ - build-automation
+ - 워크플로
+-
+ title: Auto Label Issue Based
+ description: Automatically label an issue based on the issue description
+ languages: 'TypeScript, JavaScript, Dockerfile'
+ href: Renato66/auto-label
+ tags:
+ - labels
+ - 워크플로
+ - automation
+-
+ title: Update Configured GitHub Actions to the Latest Versions
+ description: CLI tool to check whehter all your actions are up-to-date or not
+ languages: 'C#, Inno Setup, PowerSHell, Shell'
+ href: fabasoad/ghacu
+ tags:
+ - versions
+ - cli
+ - 워크플로
+-
+ title: Create Issue Branch
+ description: GitHub Action that automates the creation of issue branches
+ languages: 'JavaScript, Shell'
+ href: robvanderleek/create-issue-branch
+ tags:
+ - probot
+ - issues
+ - labels
+-
+ title: Remove old artifacts
+ description: Customize artifact cleanup
+ languages: 'JavaScript, Shell'
+ href: c-hive/gha-remove-artifacts
+ tags:
+ - artifacts
+ - 워크플로
+-
+ title: Sync Defined Files/Binaries to Wiki or External Repositories
+ description: GitHub Action to automatically sync changes to external repositories, like the wiki, for example
+ languages: 'Shell, Dockerfile'
+ href: kai-tub/external-repo-sync-action
+ tags:
+ - wiki
+ - sync
+ - 워크플로
+-
+ title: Create/Update/Delete a GitHub Wiki page based on any file
+ description: Updates your GitHub wiki by using rsync, allowing for exclusion of files and directories and actual deletion of files
+ languages: 'Shell, Dockerfile'
+ href: Andrew-Chen-Wang/github-wiki-action
+ tags:
+ - wiki
+ - docker
+ - 워크플로
+-
+ title: Prow GitHub Actions
+ description: Automation of policy enforcement, chat-ops, and automatic PR merging
+ languages: 'TypeScript, JavaScript'
+ href: jpmcb/prow-github-actions
+ tags:
+ - chat-ops
+ - prow
+ - 워크플로
+-
+ title: Check GitHub Status in your Workflow
+ description: Check GitHub Status in your workflow
+ languages: 'TypeScript, JavaScript'
+ href: crazy-max/ghaction-github-status
+ tags:
+ - 상태
+ - monitoring
+ - 워크플로
+-
+ title: Manage labels on GitHub as code
+ description: GitHub Action to manage labels (create/rename/update/delete)
+ languages: 'TypeScript, JavaScript'
+ href: crazy-max/ghaction-github-labeler
+ tags:
+ - labels
+ - 워크플로
+ - automation
+-
+ title: Distribute funding in free and open source projects
+ description: Continuous Distribution of funding to project contributors and dependencies
+ languages: 'Python, Docerfile, Shell, Ruby'
+ href: protontypes/libreselery
+ tags:
+ - sponsors
+ - funding
+ - payment
+-
+ title: Herald rules for GitHub
+ description: Add reviewers, subscribers, labels and assignees to your PR
+ languages: 'TypeScript, JavaScript'
+ href: gagoar/use-herald-action
+ tags:
+ - reviewers
+ - labels
+ - assignees
+ - 끌어오기 요청
+-
+ title: Codeowner validator
+ description: Ensures the correctness of your GitHub CODEOWNERS file, supports public and private GitHub repositories and also GitHub Enterprise installations
+ languages: 'Go, Shell, Makefile, Docerfile'
+ href: mszostok/codeowners-validator
+ tags:
+ - codeowners
+ - validate
+ - 워크플로
+-
+ title: Copybara Action
+ description: Move and transform code between repositories (ideal to maintain several repos from one monorepo)
+ languages: 'TypeScript, JavaScript, Shell'
+ href: olivr/copybara-action
+ tags:
+ - monorepo
+ - copybara
+ - 워크플로
diff --git a/translations/pt-BR/content/admin/packages/quickstart-for-configuring-minio-storage.md b/translations/pt-BR/content/admin/packages/quickstart-for-configuring-minio-storage.md
new file mode 100644
index 000000000000..e702c12faa85
--- /dev/null
+++ b/translations/pt-BR/content/admin/packages/quickstart-for-configuring-minio-storage.md
@@ -0,0 +1,133 @@
+---
+title: Início rápido para configurar o armazenamento do MinIO
+intro: 'Configure o MinIO como um provedor de armazenamento para usar {% data variables.product.prodname_registry %} na sua empresa.'
+versions:
+ enterprise-server: '>=2.22'
+---
+
+{% data reusables.package_registry.packages-ghes-release-stage %}
+
+Antes de poder habilitar e configurar {% data variables.product.prodname_registry %} em {% data variables.product.product_location_enterprise %}, você deverá preparar sua solução de armazenamento de terceiros.
+
+O MinIO oferece armazenamento de objetos com suporte para a API S3 e {% data variables.product.prodname_registry %} na sua empresa.
+
+Este início rápido mostra como configurar o MinIO usando o Docker para uso com {% data variables.product.prodname_registry %}. No entanto, você tem outras opções para gerenciar o MinIO além do Docker. Para obter mais informações sobre o MinIO, consulte a [Documentação oficial do MinIO](https://docs.min.io/).
+
+### 1. Escolha um modo MinIO para suas necessidades
+
+| Modo MinIO | Otimizado para | Infraestrutura de armazenamento necessária |
+| ---------------------------------------------------- | --------------------------- | ----------------------------------------------------- |
+| MinIO independente (em um único host) | Configuração rápida | N/A |
+| MinIO como um gateway NAS | NAS (Armazenamento de rede) | Dispositivos NAS |
+| MinIO agrupado (também denominado MinIO Distribuído) | Segurança de dados | Servidores de armazenamento em execução em um cluster |
+
+Para obter mais informações sobre suas opções, consulte [Documentação oficial do MinIO](https://docs.min.io/).
+
+### 2. Instalar, executar e efetuar o login no MinIO
+
+1. Configure suas variáveis de ambiente preferidas para o MinIO.
+
+ Estes exemplos usam `MINIO_DIR`:
+ ```shell
+ $ export MINIO_DIR=$(pwd)/minio
+ $ mkdir -p $MINIO_DIR
+ ```
+
+2. Instalar MinIO.
+
+ ```shell
+ $ docker pull minio/minio
+ ```
+ Para obter mais informações, consulte o "[Guia do início rápido do MinIO](https://docs.min.io/docs/minio-quickstart-guide)".
+
+3. Efetue o login no MinIO usando sua chave e segredo de acesso do MinIO.
+
+ {% linux %}
+ ```shell
+ $ export MINIO_ACCESS_KEY=$(cat /dev/urandom | tr -dc 'a-zA-Z0-9' | fold -w 32 | head -n 1)
+ # this one is actually a secret, so careful
+ $ export MINIO_SECRET_KEY=$(cat /dev/urandom | tr -dc 'a-zA-Z0-9' | fold -w 32 | head -n 1)
+ ```
+ {% endlinux %}
+
+ {% mac %}
+ ```shell
+ $ export MINIO_ACCESS_KEY=$(cat /dev/urandom | LC_CTYPE=C tr -dc 'a-zA-Z0-9' | fold -w 32 | head -n 1)
+ # this one is actually a secret, so careful
+ $ export MINIO_SECRET_KEY=$(cat /dev/urandom | LC_CTYPE=C tr -dc 'a-zA-Z0-9' | fold -w 32 | head -n 1)
+ ```
+ {% endmac %}
+
+ Você pode acessar suas chaves do MinIO usando as variáveis de ambiente:
+
+ ```shell
+ $ echo $MINIO_ACCESS_KEY
+ $ echo $MINIO_SECRET_KEY
+ ```
+
+4. Execute o MinIO no modo escolhido.
+
+ * Execute o MinIO usando Docker em um único host:
+
+ ```shell
+ $ docker run -p 9000:9000 \
+ -v $MINIO_DIR:/data \
+ -e "MINIO_ACCESS_KEY=$MINIO_ACCESS_KEY" \
+ -e "MINIO_SECRET_KEY=$MINIO_SECRET_KEY" \
+ minio/minio server /data
+ ```
+
+ Para obter mais informações, consulte "[Guia de início rápido do Docker do MinIO](https://docs.min.io/docs/minio-docker-quickstart-guide.html)".
+
+ * Executar o MinIO usando Docker como um gateway NAS:
+
+ Esta configuração é útil para implantações em que já existe um NAS que você deseja usar como armazenamento de backup para {% data variables.product.prodname_registry %}.
+
+ ```shell
+ $ docker run -p 9000:9000 \
+ -v $MINIO_DIR:/data \
+ -e "MINIO_ACCESS_KEY=$MINIO_ACCESS_KEY" \
+ -e "MINIO_SECRET_KEY=$MINIO_SECRET_KEY" \
+ minio/minio gateway nas /data
+ ```
+
+ Para obter mais informações, consulte "[Gateway do MinIO para NAS](https://docs.min.io/docs/minio-gateway-for-nas.html)".
+
+ * Executar o MinIO usando Docker como um cluster. Esta implantação do MinIO usa vários hosts e a codificação de eliminação do MinIO para uma proteção de dados mais forte. Para executar o MinIO em um modo de cluster, consulte o "[Guia de início rápido do MinIO distribuído](https://docs.min.io/docs/distributed-minio-quickstart-guide.html).
+
+### 3. Crie o seu bucket do MinIO para {% data variables.product.prodname_registry %}
+
+1. Instalar o cliente do MinIO.
+
+ ```shell
+ $ docker pull minio/mc
+ ```
+
+2. Criar um bucket com uma URL de host que {% data variables.product.prodname_ghe_server %} pode acessar.
+
+ * Exemplo de implantação local:
+
+ ```shell
+ $ export MC_HOST_minio="http://${MINIO_ACCESS_KEY}:${MINIO_SECRET_KEY} @localhost:9000"
+ $ docker run minio/mc BUCKET-NAME
+ ```
+
+ Este exemplo pode ser usado para MinIO independente ou MinIO como um gateway NAS.
+
+ * Exemplo de implantações agrupadas:
+
+ ```shell
+ $ export MC_HOST_minio="http://${MINIO_ACCESS_KEY}:${MINIO_SECRET_KEY} @minioclustername.example.com:9000"
+ $ docker run minio/mc mb packages
+ ```
+
+
+### Próximas etapas
+
+Para concluir a configuração de armazenamento para {% data variables.product.prodname_registry %}, você precisará copiar a URL de armazenamento do MinIO:
+
+ ```
+ echo "http://${MINIO_ACCESS_KEY}:${MINIO_SECRET_KEY}@minioclustername.example.com:9000"
+ ```
+
+Para os próximos passos, consulte "[Configurar armazenamento de terceiros para pacotes](/admin/packages/configuring-third-party-storage-for-packages).
\ No newline at end of file
diff --git a/translations/pt-BR/content/admin/release-notes.md b/translations/pt-BR/content/admin/release-notes.md
new file mode 100644
index 000000000000..fecb63f3f298
--- /dev/null
+++ b/translations/pt-BR/content/admin/release-notes.md
@@ -0,0 +1,8 @@
+---
+title: Notas de lançamento
+intro: The release notes for {{ allVersions[currentVersion].versionTitle }}.
+layout: release-notes
+versions:
+ enterprise-server: '*'
+---
+
diff --git a/translations/pt-BR/data/reusables/notifications-v2/custom-notifications-beta.md b/translations/pt-BR/data/reusables/notifications-v2/custom-notifications-beta.md
new file mode 100644
index 000000000000..dbcee80cfe1d
--- /dev/null
+++ b/translations/pt-BR/data/reusables/notifications-v2/custom-notifications-beta.md
@@ -0,0 +1,9 @@
+{% if currentVersion == "free-pro-team@latest" %}
+
+{% note %}
+
+**Note:** Custom notifications are currently in beta and subject to change.
+
+{% endnote %}
+
+{% endif %}
diff --git a/translations/pt-BR/data/reusables/repositories/administrators-can-disable-issues.md b/translations/pt-BR/data/reusables/repositories/administrators-can-disable-issues.md
new file mode 100644
index 000000000000..7562a3081c96
--- /dev/null
+++ b/translations/pt-BR/data/reusables/repositories/administrators-can-disable-issues.md
@@ -0,0 +1 @@
+Repository administrators can disable issues for a repository. Para obter mais informações, consulte "[Desabilitar problemas](/github/managing-your-work-on-github/disabling-issues)".
diff --git a/translations/pt-BR/data/reusables/search/you-can-search-globally.md b/translations/pt-BR/data/reusables/search/you-can-search-globally.md
new file mode 100644
index 000000000000..47c7190f96e6
--- /dev/null
+++ b/translations/pt-BR/data/reusables/search/you-can-search-globally.md
@@ -0,0 +1 @@
+Você pode pesquisar globalmente em todo o {% data variables.product.product_name %} ou definir o escopo da sua pesquisa para uma organização ou um repositório específico.
diff --git a/translations/ru-RU/content/actions/hosting-your-own-runners/managing-access-to-self-hosted-runners-using-groups.md b/translations/ru-RU/content/actions/hosting-your-own-runners/managing-access-to-self-hosted-runners-using-groups.md
index e0d1e320cdd1..d1641858552a 100644
--- a/translations/ru-RU/content/actions/hosting-your-own-runners/managing-access-to-self-hosted-runners-using-groups.md
+++ b/translations/ru-RU/content/actions/hosting-your-own-runners/managing-access-to-self-hosted-runners-using-groups.md
@@ -33,7 +33,7 @@ All organizations have a single default self-hosted runner group. Organizations
Self-hosted runners are automatically assigned to the default group when created, and can only be members of one group at a time. You can move a runner from the default group to any group you create.
-When creating a group, you must choose a policy that defines which repositories have access to the runner group. You can configure a runner group to be accessible to a specific list of repositories, all private repositories, or all repositories in the organization.
+When creating a group, you must choose a policy that defines which repositories have access to the runner group.
{% data reusables.organizations.navigate-to-org %}
{% data reusables.organizations.org_settings %}
@@ -41,9 +41,19 @@ When creating a group, you must choose a policy that defines which repositories
1. In the **Self-hosted runners** section, click **Add new**, and then **New group**.

-1. Enter a name for your runner group, and select an access policy from the **Repository access** dropdown list.
+1. Enter a name for your runner group, and assign a policy for repository access.
- 
+ {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %} You can configure a runner group to be accessible to a specific list of repositories, or to all repositories in the organization. By default, public repositories can't access runners in a runner group, but you can use the **Allow public repositories** option to override this.{% else if currentVersion == "enterprise-server@2.22"%}You can configure a runner group to be accessible to a specific list of repositories, all private repositories, or all repositories in the organization.{% endif %}
+
+ {% warning %}
+
+ **Warnung**
+ {% indented_data_reference site.data.reusables.github-actions.self-hosted-runner-security spaces=3 %}
+ Weitere Informationen findest Du unter „[Informationen zu selbst-gehosteten Runnern](/actions/hosting-your-own-runners/about-self-hosted-runners#self-hosted-runner-security-with-public-repositories)“.
+
+ {% endwarning %}
+
+ 
1. Click **Save group** to create the group and apply the policy.
### Creating a self-hosted runner group for an enterprise
@@ -52,7 +62,7 @@ Enterprises can add their self-hosted runners to groups for access management. E
Self-hosted runners are automatically assigned to the default group when created, and can only be members of one group at a time. You can assign the runner to a specific group during the registration process, or you can later move the runner from the default group to a custom group.
-When creating a group, you must choose a policy that grants access to all organizations in the enterprise or choose specific organizations.
+When creating a group, you must choose a policy that defines which organizations have access to the runner group.
{% data reusables.enterprise-accounts.access-enterprise %}
{% data reusables.enterprise-accounts.policies-tab %}
@@ -61,7 +71,17 @@ When creating a group, you must choose a policy that grants access to all organi
1. Click **Add new**, and then **New group**.

-1. Enter a name for your runner group, and select an access policy from the **Organization access** dropdown list.
+1. Enter a name for your runner group, and assign a policy for organization access.
+
+ {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %} You can configure a runner group to be accessible to a specific list of organizations, or all organizations in the enterprise. By default, public repositories can't access runners in a runner group, but you can use the **Allow public repositories** option to override this.{% else if currentVersion == "enterprise-server@2.22"%}You can configure a runner group to be accessible to all organizations in the enterprise or choose specific organizations.{% endif %}
+
+ {% warning %}
+
+ **Warnung**
+ {% indented_data_reference site.data.reusables.github-actions.self-hosted-runner-security spaces=3 %}
+ Weitere Informationen findest Du unter „[Informationen zu selbst-gehosteten Runnern](/actions/hosting-your-own-runners/about-self-hosted-runners#self-hosted-runner-security-with-public-repositories)“.
+
+ {% endwarning %}

1. Click **Save group** to create the group and apply the policy.
@@ -89,3 +109,4 @@ Self-hosted runners are automatically returned to the default group when their g
1. To remove the group, click **Remove group**. 
1. Review the confirmation prompts, and click **Remove this runner group**.
+
diff --git a/translations/ru-RU/content/actions/reference/events-that-trigger-workflows.md b/translations/ru-RU/content/actions/reference/events-that-trigger-workflows.md
index 61dd7738cba1..5de868c0aeb6 100644
--- a/translations/ru-RU/content/actions/reference/events-that-trigger-workflows.md
+++ b/translations/ru-RU/content/actions/reference/events-that-trigger-workflows.md
@@ -572,6 +572,8 @@ on:
{% data reusables.developer-site.pull_request_forked_repos_link %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %}
+
#### `pull_request_target`
This event is similar to `pull_request`, except that it runs in the context of the base repository of the pull request, rather than in the merge commit. This means that you can more safely make your secrets available to the workflows triggered by the pull request, because only workflows defined in the commit on the base repository are run. For example, this event allows you to create workflows that label and comment on pull requests, based on the contents of the event payload.
@@ -589,6 +591,8 @@ on: pull_request_target
types: [assigned, opened, synchronize, reopened]
```
+{% endif %}
+
#### `отправка`
{% note %}
@@ -689,6 +693,8 @@ on:
types: [started]
```
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %}
+
#### `workflow_run`
{% data reusables.webhooks.workflow_run_desc %}
@@ -711,6 +717,8 @@ on:
- requested
```
+{% endif %}
+
### Triggering new workflows using a personal access token
{% data reusables.github-actions.actions-do-not-trigger-workflows %} For more information, see "[Authenticating with the GITHUB_TOKEN](/actions/configuring-and-managing-workflows/authenticating-with-the-github_token)."
diff --git a/translations/ru-RU/content/actions/reference/workflow-syntax-for-github-actions.md b/translations/ru-RU/content/actions/reference/workflow-syntax-for-github-actions.md
index b68997495c83..b05cc41dbca4 100644
--- a/translations/ru-RU/content/actions/reference/workflow-syntax-for-github-actions.md
+++ b/translations/ru-RU/content/actions/reference/workflow-syntax-for-github-actions.md
@@ -876,6 +876,37 @@ strategy:
{% endnote %}
+##### Using environment variables in a matrix
+
+You can add custom environment variables for each test combination by using `include` with `env`. You can then refer to the custom environment variables in a later step.
+
+In this example, the matrix entries for `node-version` are each configured to use different values for the `site` and `datacenter` environment variables. The `Echo site details` step then uses {% raw %}`env: ${{ matrix.env }}`{% endraw %} to refer to the custom variables:
+
+{% raw %}
+```yaml
+name: Node.js CI
+on: [push]
+jobs:
+ build:
+ runs-on: ubuntu-latest
+ strategy:
+ matrix:
+ include:
+ - node-version: 10.x
+ site: "prod"
+ datacenter: "site-a"
+ - node-version: 12.x
+ site: "dev"
+ datacenter: "site-b"
+ steps:
+ - name: Echo site details
+ env:
+ SITE: ${{ matrix.site }}
+ DATACENTER: ${{ matrix.datacenter }}
+ run: echo $SITE $DATACENTER
+```
+{% endraw %}
+
### **`jobs..strategy.fail-fast`**
When set to `true`, {% data variables.product.prodname_dotcom %} cancels all in-progress jobs if any `matrix` job fails. Default: `true`
diff --git a/translations/ru-RU/content/admin/configuration/command-line-utilities.md b/translations/ru-RU/content/admin/configuration/command-line-utilities.md
index c03d65e8380f..495c2290d9b6 100644
--- a/translations/ru-RU/content/admin/configuration/command-line-utilities.md
+++ b/translations/ru-RU/content/admin/configuration/command-line-utilities.md
@@ -84,7 +84,7 @@ Allows you to find the uuid of your node in `cluster.conf`.
Allows you to exempt a list of users from API rate limits. For more information, see "[Resources in the REST API](/rest/overview/resources-in-the-rest-api#rate-limiting)."
``` shell
-$ ghe-config app.github.rate_limiting_exempt_users "hubot github-actions"
+$ ghe-config app.github.rate-limiting-exempt-users "hubot github-actions"
# Exempts the users hubot and github-actions from rate limits
```
{% endif %}
diff --git a/translations/ru-RU/content/admin/enterprise-management/configuring-high-availability-replication-for-a-cluster.md b/translations/ru-RU/content/admin/enterprise-management/configuring-high-availability-replication-for-a-cluster.md
index 40895c206992..2c873436256a 100644
--- a/translations/ru-RU/content/admin/enterprise-management/configuring-high-availability-replication-for-a-cluster.md
+++ b/translations/ru-RU/content/admin/enterprise-management/configuring-high-availability-replication-for-a-cluster.md
@@ -57,32 +57,36 @@ Before you define a secondary datacenter for your passive nodes, ensure that you
mysql-master = HOSTNAME
redis-master = HOSTNAME
primary-datacenter = default
- ```
+ ```
- Optionally, change the name of the primary datacenter to something more descriptive or accurate by editing the value of `primary-datacenter`.
4. {% data reusables.enterprise_clustering.configuration-file-heading %} Under each node's heading, add a new key-value pair to assign the node to a datacenter. Use the same value as `primary-datacenter` from step 3 above. For example, if you want to use the default name (`default`), add the following key-value pair to the section for each node.
- datacenter = default
+ ```
+ datacenter = default
+ ```
When you're done, the section for each node in the cluster configuration file should look like the following example. {% data reusables.enterprise_clustering.key-value-pair-order-irrelevant %}
- ```shell
- [cluster "HOSTNAME"]
- datacenter = default
- hostname = HOSTNAME
- ipv4 = IP ADDRESS
+ ```shell
+ [cluster "HOSTNAME"]
+ datacenter = default
+ hostname = HOSTNAME
+ ipv4 = IP ADDRESS
+ ...
...
- ...
- ```
+ ```
- {% note %}
+ {% note %}
- **Note**: If you changed the name of the primary datacenter in step 3, find the `consul-datacenter` key-value pair in the section for each node and change the value to the renamed primary datacenter. For example, if you named the primary datacenter `primary`, use the following key-value pair for each node.
+ **Note**: If you changed the name of the primary datacenter in step 3, find the `consul-datacenter` key-value pair in the section for each node and change the value to the renamed primary datacenter. For example, if you named the primary datacenter `primary`, use the following key-value pair for each node.
- consul-datacenter = primary
+ ```
+ consul-datacenter = primary
+ ```
- {% endnote %}
+ {% endnote %}
{% data reusables.enterprise_clustering.apply-configuration %}
@@ -103,31 +107,37 @@ For an example configuration, see "[Example configuration](#example-configuratio
1. For each node in your cluster, provision a matching virtual machine with identical specifications, running the same version of {% data variables.product.prodname_ghe_server %}. Note the IPv4 address and hostname for each new cluster node. For more information, see "[Prerequisites](#prerequisites)."
- {% note %}
+ {% note %}
- **Note**: If you're reconfiguring high availability after a failover, you can use the old nodes from the primary datacenter instead.
+ **Note**: If you're reconfiguring high availability after a failover, you can use the old nodes from the primary datacenter instead.
- {% endnote %}
+ {% endnote %}
{% data reusables.enterprise_clustering.ssh-to-a-node %}
3. Back up your existing cluster configuration.
-
- cp /data/user/common/cluster.conf ~/$(date +%Y-%m-%d)-cluster.conf.backup
+
+ ```
+ cp /data/user/common/cluster.conf ~/$(date +%Y-%m-%d)-cluster.conf.backup
+ ```
4. Create a copy of your existing cluster configuration file in a temporary location, like _/home/admin/cluster-passive.conf_. Delete unique key-value pairs for IP addresses (`ipv*`), UUIDs (`uuid`), and public keys for WireGuard (`wireguard-pubkey`).
-
- grep -Ev "(?:|ipv|uuid|vpn|wireguard\-pubkey)" /data/user/common/cluster.conf > ~/cluster-passive.conf
+
+ ```
+ grep -Ev "(?:|ipv|uuid|vpn|wireguard\-pubkey)" /data/user/common/cluster.conf > ~/cluster-passive.conf
+ ```
5. Remove the `[cluster]` section from the temporary cluster configuration file that you copied in the previous step.
-
- git config -f ~/cluster-passive.conf --remove-section cluster
+
+ ```
+ git config -f ~/cluster-passive.conf --remove-section cluster
+ ```
6. Decide on a name for the secondary datacenter where you provisioned your passive nodes, then update the temporary cluster configuration file with the new datacenter name. Replace `SECONDARY` with the name you choose.
```shell
- sed -i 's/datacenter = default/datacenter = SECONDARY/g' ~/cluster-passive.conf
- ```
+ sed -i 's/datacenter = default/datacenter = SECONDARY/g' ~/cluster-passive.conf
+ ```
7. Decide on a pattern for the passive nodes' hostnames.
@@ -140,7 +150,7 @@ For an example configuration, see "[Example configuration](#example-configuratio
8. Open the temporary cluster configuration file from step 3 in a text editor. For example, you can use Vim.
```shell
- sudo vim ~/cluster-passive.conf
+ sudo vim ~/cluster-passive.conf
```
9. In each section within the temporary cluster configuration file, update the node's configuration. {% data reusables.enterprise_clustering.configuration-file-heading %}
@@ -150,37 +160,37 @@ For an example configuration, see "[Example configuration](#example-configuratio
- Add a new key-value pair, `replica = enabled`.
```shell
- [cluster "NEW PASSIVE NODE HOSTNAME"]
+ [cluster "NEW PASSIVE NODE HOSTNAME"]
+ ...
+ hostname = NEW PASSIVE NODE HOSTNAME
+ ipv4 = NEW PASSIVE NODE IPV4 ADDRESS
+ replica = enabled
+ ...
...
- hostname = NEW PASSIVE NODE HOSTNAME
- ipv4 = NEW PASSIVE NODE IPV4 ADDRESS
- replica = enabled
- ...
- ...
```
10. Append the contents of the temporary cluster configuration file that you created in step 4 to the active configuration file.
```shell
- cat ~/cluster-passive.conf >> /data/user/common/cluster.conf
- ```
+ cat ~/cluster-passive.conf >> /data/user/common/cluster.conf
+ ```
11. Designate the primary MySQL and Redis nodes in the secondary datacenter. Replace `REPLICA MYSQL PRIMARY HOSTNAME` and `REPLICA REDIS PRIMARY HOSTNAME` with the hostnames of the passives node that you provisioned to match your existing MySQL and Redis primaries.
```shell
- git config -f /data/user/common/cluster.conf cluster.mysql-master-replica REPLICA MYSQL PRIMARY HOSTNAME
- git config -f /data/user/common/cluster.conf cluster.redis-master-replica REPLICA REDIS PRIMARY HOSTNAME
- ```
+ git config -f /data/user/common/cluster.conf cluster.mysql-master-replica REPLICA MYSQL PRIMARY HOSTNAME
+ git config -f /data/user/common/cluster.conf cluster.redis-master-replica REPLICA REDIS PRIMARY HOSTNAME
+ ```
12. Enable MySQL to fail over automatically when you fail over to the passive replica nodes.
```shell
- git config -f /data/user/common/cluster.conf cluster.mysql-auto-failover true
+ git config -f /data/user/common/cluster.conf cluster.mysql-auto-failover true
```
- {% warning %}
+ {% warning %}
- **Warning**: Review your cluster configuration file before proceeding.
+ **Warning**: Review your cluster configuration file before proceeding.
- In the top-level `[cluster]` section, ensure that the values for `mysql-master-replica` and `redis-master-replica` are the correct hostnames for the passive nodes in the secondary datacenter that will serve as the MySQL and Redis primaries after a failover.
- In each section for an active node named `[cluster "ACTIVE NODE HOSTNAME"]`, double-check the following key-value pairs.
@@ -194,9 +204,9 @@ For an example configuration, see "[Example configuration](#example-configuratio
- `replica` should be configured as `enabled`.
- Take the opportunity to remove sections for offline nodes that are no longer in use.
- To review an example configuration, see "[Example configuration](#example-configuration)."
+ To review an example configuration, see "[Example configuration](#example-configuration)."
- {% endwarning %}
+ {% endwarning %}
13. Initialize the new cluster configuration. {% data reusables.enterprise.use-a-multiplexer %}
@@ -207,7 +217,7 @@ For an example configuration, see "[Example configuration](#example-configuratio
14. After the initialization finishes, {% data variables.product.prodname_ghe_server %} displays the following message.
```shell
- Finished cluster initialization
+ Finished cluster initialization
```
{% data reusables.enterprise_clustering.apply-configuration %}
@@ -293,20 +303,28 @@ Initial replication between the active and passive nodes in your cluster takes t
You can monitor the progress on any node in the cluster, using command-line tools available via the {% data variables.product.prodname_ghe_server %} administrative shell. For more information about the administrative shell, see "[Accessing the administrative shell (SSH)](/enterprise/admin/configuration/accessing-the-administrative-shell-ssh)."
- Monitor replication of databases:
-
- /usr/local/share/enterprise/ghe-cluster-status-mysql
+
+ ```
+ /usr/local/share/enterprise/ghe-cluster-status-mysql
+ ```
- Monitor replication of repository and Gist data:
-
- ghe-spokes status
+
+ ```
+ ghe-spokes status
+ ```
- Monitor replication of attachment and LFS data:
-
- ghe-storage replication-status
+
+ ```
+ ghe-storage replication-status
+ ```
- Monitor replication of Pages data:
-
- ghe-dpages replication-status
+
+ ```
+ ghe-dpages replication-status
+ ```
You can use `ghe-cluster-status` to review the overall health of your cluster. For more information, see "[Command-line utilities](/enterprise/admin/configuration/command-line-utilities#ghe-cluster-status)."
diff --git a/translations/ru-RU/content/admin/enterprise-management/increasing-storage-capacity.md b/translations/ru-RU/content/admin/enterprise-management/increasing-storage-capacity.md
index bb41f8c6021a..1b8a6164c8e9 100644
--- a/translations/ru-RU/content/admin/enterprise-management/increasing-storage-capacity.md
+++ b/translations/ru-RU/content/admin/enterprise-management/increasing-storage-capacity.md
@@ -20,6 +20,8 @@ As more users join {% data variables.product.product_location %}, you may need t
{% endnote %}
+#### Minimum requirements
+
{% data reusables.enterprise_installation.hardware-rec-table %}
### Increasing the data partition size
diff --git a/translations/ru-RU/content/admin/installation/installing-github-enterprise-server-on-google-cloud-platform.md b/translations/ru-RU/content/admin/installation/installing-github-enterprise-server-on-google-cloud-platform.md
index fbb6ad02fda0..db7f2444cdf1 100644
--- a/translations/ru-RU/content/admin/installation/installing-github-enterprise-server-on-google-cloud-platform.md
+++ b/translations/ru-RU/content/admin/installation/installing-github-enterprise-server-on-google-cloud-platform.md
@@ -26,14 +26,14 @@ Before launching {% data variables.product.product_location %} on Google Cloud P
{% data variables.product.prodname_ghe_server %} is supported on the following Google Compute Engine (GCE) machine types. For more information, see [the Google Cloud Platform machine types article](https://cloud.google.com/compute/docs/machine-types).
-| | High-memory |
-| | ------------- |
-| | n1-highmem-4 |
-| | n1-highmem-8 |
-| | n1-highmem-16 |
-| | n1-highmem-32 |
-| | n1-highmem-64 |
-| | n1-highmem-96 |
+| High-memory |
+| ------------- |
+| n1-highmem-4 |
+| n1-highmem-8 |
+| n1-highmem-16 |
+| n1-highmem-32 |
+| n1-highmem-64 |
+| n1-highmem-96 |
#### Recommended machine types
@@ -54,7 +54,7 @@ Based on your user license count, we recommend these machine types.
1. Using the [gcloud compute](https://cloud.google.com/compute/docs/gcloud-compute/) command-line tool, list the public {% data variables.product.prodname_ghe_server %} images:
```shell
$ gcloud compute images list --project github-enterprise-public --no-standard-images
- ```
+ ```
2. Take note of the image name for the latest GCE image of {% data variables.product.prodname_ghe_server %}.
@@ -63,18 +63,18 @@ Based on your user license count, we recommend these machine types.
GCE virtual machines are created as a member of a network, which has a firewall. For the network associated with the {% data variables.product.prodname_ghe_server %} VM, you'll need to configure the firewall to allow the required ports listed in the table below. For more information about firewall rules on Google Cloud Platform, see the Google guide "[Firewall Rules Overview](https://cloud.google.com/vpc/docs/firewalls)."
1. Using the gcloud compute command-line tool, create the network. For more information, see "[gcloud compute networks create](https://cloud.google.com/sdk/gcloud/reference/compute/networks/create)" in the Google documentation.
- ```shell
- $ gcloud compute networks create NETWORK-NAME --subnet-mode auto
- ```
+ ```shell
+ $ gcloud compute networks create NETWORK-NAME --subnet-mode auto
+ ```
2. Create a firewall rule for each of the ports in the table below. For more information, see "[gcloud compute firewall-rules](https://cloud.google.com/sdk/gcloud/reference/compute/firewall-rules/)" in the Google documentation.
- ```shell
- $ gcloud compute firewall-rules create RULE-NAME \
- --network NETWORK-NAME \
- --allow tcp:22,tcp:25,tcp:80,tcp:122,udp:161,tcp:443,udp:1194,tcp:8080,tcp:8443,tcp:9418,icmp
- ```
- This table identifies the required ports and what each port is used for.
+ ```shell
+ $ gcloud compute firewall-rules create RULE-NAME \
+ --network NETWORK-NAME \
+ --allow tcp:22,tcp:25,tcp:80,tcp:122,udp:161,tcp:443,udp:1194,tcp:8080,tcp:8443,tcp:9418,icmp
+ ```
+ This table identifies the required ports and what each port is used for.
- {% data reusables.enterprise_installation.necessary_ports %}
+ {% data reusables.enterprise_installation.necessary_ports %}
### Allocating a static IP and assigning it to the VM
@@ -87,21 +87,21 @@ In production High Availability configurations, both primary and replica applian
To create the {% data variables.product.prodname_ghe_server %} instance, you'll need to create a GCE instance with your {% data variables.product.prodname_ghe_server %} image and attach an additional storage volume for your instance data. For more information, see "[Hardware considerations](#hardware-considerations)."
1. Using the gcloud compute command-line tool, create a data disk to use as an attached storage volume for your instance data, and configure the size based on your user license count. For more information, see "[gcloud compute disks create](https://cloud.google.com/sdk/gcloud/reference/compute/disks/create)" in the Google documentation.
- ```shell
- $ gcloud compute disks create DATA-DISK-NAME --size DATA-DISK-SIZE --type DATA-DISK-TYPE --zone ZONE
- ```
+ ```shell
+ $ gcloud compute disks create DATA-DISK-NAME --size DATA-DISK-SIZE --type DATA-DISK-TYPE --zone ZONE
+ ```
2. Then create an instance using the name of the {% data variables.product.prodname_ghe_server %} image you selected, and attach the data disk. For more information, see "[gcloud compute instances create](https://cloud.google.com/sdk/gcloud/reference/compute/instances/create)" in the Google documentation.
- ```shell
- $ gcloud compute instances create INSTANCE-NAME \
- --machine-type n1-standard-8 \
- --image GITHUB-ENTERPRISE-IMAGE-NAME \
- --disk name=DATA-DISK-NAME \
- --metadata serial-port-enable=1 \
- --zone ZONE \
- --network NETWORK-NAME \
- --image-project github-enterprise-public
- ```
+ ```shell
+ $ gcloud compute instances create INSTANCE-NAME \
+ --machine-type n1-standard-8 \
+ --image GITHUB-ENTERPRISE-IMAGE-NAME \
+ --disk name=DATA-DISK-NAME \
+ --metadata serial-port-enable=1 \
+ --zone ZONE \
+ --network NETWORK-NAME \
+ --image-project github-enterprise-public
+ ```
### Configuring the instance
diff --git a/translations/ru-RU/content/admin/overview/about-enterprise-accounts.md b/translations/ru-RU/content/admin/overview/about-enterprise-accounts.md
index 3d1804f81f01..02c92ad31de7 100644
--- a/translations/ru-RU/content/admin/overview/about-enterprise-accounts.md
+++ b/translations/ru-RU/content/admin/overview/about-enterprise-accounts.md
@@ -1,26 +1,31 @@
---
title: About enterprise accounts
-intro: 'With {% data variables.product.prodname_ghe_server %}, you can create an enterprise account to give administrators a single point of visibility and management for their billing and license usage.'
+intro: 'With {% data variables.product.product_name %}, you can use an enterprise account to give administrators a single point of visibility and management{% if enterpriseServerVersions contains currentVersion %} for billing and license usage{% endif %}.'
redirect_from:
- /enterprise/admin/installation/about-enterprise-accounts
- /enterprise/admin/overview/about-enterprise-accounts
versions:
- enterprise-server: '*'
+ enterprise-server: '>=2.20'
+ github-ae: '*'
---
-### About enterprise accounts on {% data variables.product.prodname_ghe_server %}
+### About enterprise accounts on {% data variables.product.product_name %}
-An enterprise account allows you to manage multiple {% data variables.product.prodname_dotcom %} organizations and {% data variables.product.prodname_ghe_server %} instances. Your enterprise account must have a handle, like an organization or personal account on {% data variables.product.prodname_dotcom %}. Enterprise administrators can manage settings and preferences, like:
+An enterprise account allows you to manage multiple organizations{% if enterpriseServerVersions contains currentVersion %} and {% data variables.product.prodname_ghe_server %} instances{% else %} on {% data variables.product.product_name %}{% endif %}. Your enterprise account must have a handle, like an organization or personal account on {% data variables.product.prodname_dotcom %}. Enterprise administrators can manage settings and preferences, like:
-- Member access and management (organization members, outside collaborators)
-- Billing and usage ({% data variables.product.prodname_ghe_server %} instances, user licenses, {% data variables.large_files.product_name_short %} packs)
-- Security (single sign-on, two factor authentication)
-- Requests and support bundle sharing with {% data variables.contact.enterprise_support %}
+- Member access and management (organization members, outside collaborators){% if enterpriseServerVersions contains currentVersion %}
+- Billing and usage ({% data variables.product.prodname_ghe_server %} instances, user licenses, {% data variables.large_files.product_name_short %} packs){% endif %}
+- Security{% if enterpriseServerVersions contains currentVersion %}(single sign-on, two factor authentication)
+- Requests {% if enterpriseServerVersions contains currentVersion %}and support bundle sharing {% endif %}with {% data variables.contact.enterprise_support %}{% endif %}
-{% data reusables.enterprise-accounts.enterprise-accounts-billing %}
+{% if enterpriseServerVersions contains currentVersion %}{% data reusables.enterprise-accounts.enterprise-accounts-billing %} For more information about managing your {% data variables.product.prodname_ghe_cloud %} subscription, see "[Viewing the subscription and usage for your enterprise account](/articles/viewing-the-subscription-and-usage-for-your-enterprise-account)." {% endif %}For more information about managing your {% data variables.product.product_name %} billing settings, see "[Managing billing for your enterprise](/admin/overview/managing-billing-for-your-enterprise)."
+
+{% if enterpriseServerVersions contains currentVersion %}
For more information about the differences between {% data variables.product.prodname_ghe_cloud %} and {% data variables.product.prodname_ghe_server %}, see "[{% data variables.product.prodname_dotcom %}'s products](/articles/githubs-products)." To upgrade to {% data variables.product.prodname_enterprise %} or to get started with an enterprise account, contact {% data variables.contact.contact_enterprise_sales %}.
### Managing {% data variables.product.prodname_ghe_server %} licenses linked to your enterprise account
{% data reusables.enterprise-accounts.admin-managing-licenses %}
+
+{% endif %}
diff --git a/translations/ru-RU/content/admin/packages/configuring-third-party-storage-for-packages.md b/translations/ru-RU/content/admin/packages/configuring-third-party-storage-for-packages.md
index 2a7e32e8c90d..82e18d48a737 100644
--- a/translations/ru-RU/content/admin/packages/configuring-third-party-storage-for-packages.md
+++ b/translations/ru-RU/content/admin/packages/configuring-third-party-storage-for-packages.md
@@ -13,7 +13,7 @@ versions:
{% data variables.product.prodname_registry %} on {% data variables.product.prodname_ghe_server %} uses external blob storage to store your packages. The amount of storage required depends on your usage of {% data variables.product.prodname_registry %}.
-At this time, {% data variables.product.prodname_registry %} supports blob storage with Amazon Web Services (AWS) S3. MinIO is also supported, but configuration is not currently implemented in the {% data variables.product.product_name %} interface. You can use MinIO for storage by following the instructions for AWS S3, entering the analogous information for your MinIO configuration.
+At this time, {% data variables.product.prodname_registry %} supports blob storage with Amazon Web Services (AWS) S3. MinIO is also supported, but configuration is not currently implemented in the {% data variables.product.product_name %} interface. You can use MinIO for storage by following the instructions for AWS S3, entering the analogous information for your MinIO configuration. Before configuring third-party storage for {% data variables.product.prodname_registry %} on {% data variables.product.prodname_dotcom %}, you must set up a bucket with your third-party storage provider. For more information on installing and running a MinIO bucket to use with {% data variables.product.prodname_registry %}, see the "[Quickstart for configuring MinIO storage](/admin/packages/quickstart-for-configuring-minio-storage)."
For the best experience, we recommend using a dedicated bucket for {% data variables.product.prodname_registry %}, separate from the bucket you use for {% data variables.product.prodname_actions %} storage.
diff --git a/translations/ru-RU/content/admin/packages/index.md b/translations/ru-RU/content/admin/packages/index.md
index 2c1a7b4d0c1f..f73f90725995 100644
--- a/translations/ru-RU/content/admin/packages/index.md
+++ b/translations/ru-RU/content/admin/packages/index.md
@@ -10,5 +10,6 @@ versions:
{% data reusables.package_registry.packages-ghes-release-stage %}
{% link_with_intro /enabling-github-packages-for-your-enterprise %}
+{% link_with_intro /quickstart-for-configuring-minio-storage %}
{% link_with_intro /configuring-packages-support-for-your-enterprise %}
{% link_with_intro /configuring-third-party-storage-for-packages %}
diff --git a/translations/ru-RU/content/admin/packages/quickstart-for-configuring-minio-storage.md b/translations/ru-RU/content/admin/packages/quickstart-for-configuring-minio-storage.md
new file mode 100644
index 000000000000..ab927406fd4e
--- /dev/null
+++ b/translations/ru-RU/content/admin/packages/quickstart-for-configuring-minio-storage.md
@@ -0,0 +1,133 @@
+---
+title: Quickstart for configuring MinIO storage
+intro: 'Set up MinIO as a storage provider for using {% data variables.product.prodname_registry %} on your enterprise.'
+versions:
+ enterprise-server: '>=2.22'
+---
+
+{% data reusables.package_registry.packages-ghes-release-stage %}
+
+Before you can enable and configure {% data variables.product.prodname_registry %} on {% data variables.product.product_location_enterprise %}, you need to prepare your third-party storage solution.
+
+MinIO offers object storage with support for the S3 API and {% data variables.product.prodname_registry %} on your enterprise.
+
+This quickstart shows you how to set up MinIO using Docker for use with {% data variables.product.prodname_registry %} but you have other options for managing MinIO besides Docker. For more information about MinIO, see the official [MinIO docs](https://docs.min.io/).
+
+### 1. Choose a MinIO mode for your needs
+
+| MinIO mode | Optimized for | Storage infrastructure required |
+| ----------------------------------------------- | ------------------------------ | ------------------------------------ |
+| Standalone MinIO (on a single host) | Fast setup | Нет |
+| MinIO as a NAS gateway | NAS (Network-attached storage) | NAS devices |
+| Clustered MinIO (also called Distributed MinIO) | Data security | Storage servers running in a cluster |
+
+For more information about your options, see the official [MinIO docs](https://docs.min.io/).
+
+### 2. Install, run, and sign in to MinIO
+
+1. Set up your preferred environment variables for MinIO.
+
+ These examples use `MINIO_DIR`:
+ ```shell
+ $ export MINIO_DIR=$(pwd)/minio
+ $ mkdir -p $MINIO_DIR
+ ```
+
+2. Install MinIO.
+
+ ```shell
+ $ docker pull minio/minio
+ ```
+ For more information, see the official "[MinIO Quickstart Guide](https://docs.min.io/docs/minio-quickstart-guide)."
+
+3. Sign in to MinIO using your MinIO access key and secret.
+
+ {% linux %}
+ ```shell
+ $ export MINIO_ACCESS_KEY=$(cat /dev/urandom | tr -dc 'a-zA-Z0-9' | fold -w 32 | head -n 1)
+ # this one is actually a secret, so careful
+ $ export MINIO_SECRET_KEY=$(cat /dev/urandom | tr -dc 'a-zA-Z0-9' | fold -w 32 | head -n 1)
+ ```
+ {% endlinux %}
+
+ {% mac %}
+ ```shell
+ $ export MINIO_ACCESS_KEY=$(cat /dev/urandom | LC_CTYPE=C tr -dc 'a-zA-Z0-9' | fold -w 32 | head -n 1)
+ # this one is actually a secret, so careful
+ $ export MINIO_SECRET_KEY=$(cat /dev/urandom | LC_CTYPE=C tr -dc 'a-zA-Z0-9' | fold -w 32 | head -n 1)
+ ```
+ {% endmac %}
+
+ You can access your MinIO keys using the environment variables:
+
+ ```shell
+ $ echo $MINIO_ACCESS_KEY
+ $ echo $MINIO_SECRET_KEY
+ ```
+
+4. Run MinIO in your chosen mode.
+
+ * Run MinIO using Docker on a single host:
+
+ ```shell
+ $ docker run -p 9000:9000 \
+ -v $MINIO_DIR:/data \
+ -e "MINIO_ACCESS_KEY=$MINIO_ACCESS_KEY" \
+ -e "MINIO_SECRET_KEY=$MINIO_SECRET_KEY" \
+ minio/minio server /data
+ ```
+
+ For more information, see "[MinIO Docker Quickstart guide](https://docs.min.io/docs/minio-docker-quickstart-guide.html)."
+
+ * Run MinIO using Docker as a NAS gateway:
+
+ This setup is useful for deployments where there is already a NAS you want to use as the backup storage for {% data variables.product.prodname_registry %}.
+
+ ```shell
+ $ docker run -p 9000:9000 \
+ -v $MINIO_DIR:/data \
+ -e "MINIO_ACCESS_KEY=$MINIO_ACCESS_KEY" \
+ -e "MINIO_SECRET_KEY=$MINIO_SECRET_KEY" \
+ minio/minio gateway nas /data
+ ```
+
+ For more information, see "[MinIO Gateway for NAS](https://docs.min.io/docs/minio-gateway-for-nas.html)."
+
+ * Run MinIO using Docker as a cluster. This MinIO deployment uses several hosts and MinIO's erasure coding for the strongest data protection. To run MinIO in a cluster mode, see the "[Distributed MinIO Quickstart Guide](https://docs.min.io/docs/distributed-minio-quickstart-guide.html).
+
+### 3. Create your MinIO bucket for {% data variables.product.prodname_registry %}
+
+1. Install the MinIO client.
+
+ ```shell
+ $ docker pull minio/mc
+ ```
+
+2. Create a bucket with a host URL that {% data variables.product.prodname_ghe_server %} can access.
+
+ * Local deployments example:
+
+ ```shell
+ $ export MC_HOST_minio="http://${MINIO_ACCESS_KEY}:${MINIO_SECRET_KEY} @localhost:9000"
+ $ docker run minio/mc BUCKET-NAME
+ ```
+
+ This example can be used for MinIO standalone or MinIO as a NAS gateway.
+
+ * Clustered deployments example:
+
+ ```shell
+ $ export MC_HOST_minio="http://${MINIO_ACCESS_KEY}:${MINIO_SECRET_KEY} @minioclustername.example.com:9000"
+ $ docker run minio/mc mb packages
+ ```
+
+
+### Дальнейшие шаги
+
+To finish configuring storage for {% data variables.product.prodname_registry %}, you'll need to copy the MinIO storage URL:
+
+ ```
+ echo "http://${MINIO_ACCESS_KEY}:${MINIO_SECRET_KEY}@minioclustername.example.com:9000"
+ ```
+
+For the next steps, see "[Configuring third-party storage for packages](/admin/packages/configuring-third-party-storage-for-packages)."
\ No newline at end of file
diff --git a/translations/ru-RU/content/admin/policies/creating-a-pre-receive-hook-environment.md b/translations/ru-RU/content/admin/policies/creating-a-pre-receive-hook-environment.md
index 0bb35fec73bd..b37e4a0e7ba0 100644
--- a/translations/ru-RU/content/admin/policies/creating-a-pre-receive-hook-environment.md
+++ b/translations/ru-RU/content/admin/policies/creating-a-pre-receive-hook-environment.md
@@ -21,10 +21,10 @@ You can use a Linux container management tool to build a pre-receive hook enviro
{% data reusables.linux.ensure-docker %}
2. Create the file `Dockerfile.alpine-3.3` that contains this information:
- ```
- FROM gliderlabs/alpine:3.3
- RUN apk add --no-cache git bash
- ```
+ ```
+ FROM gliderlabs/alpine:3.3
+ RUN apk add --no-cache git bash
+ ```
3. From the working directory that contains `Dockerfile.alpine-3.3`, build an image:
```shell
@@ -36,37 +36,37 @@ You can use a Linux container management tool to build a pre-receive hook enviro
> ---> Using cache
> ---> 0250ab3be9c5
> Successfully built 0250ab3be9c5
- ```
+ ```
4. Create a container:
```shell
$ docker create --name pre-receive.alpine-3.3 pre-receive.alpine-3.3 /bin/true
- ```
+ ```
5. Export the Docker container to a `gzip` compressed `tar` file:
```shell
$ docker export pre-receive.alpine-3.3 | gzip > alpine-3.3.tar.gz
- ```
+ ```
- This file `alpine-3.3.tar.gz` is ready to be uploaded to the {% data variables.product.prodname_ghe_server %} appliance.
+ This file `alpine-3.3.tar.gz` is ready to be uploaded to the {% data variables.product.prodname_ghe_server %} appliance.
### Creating a pre-receive hook environment using chroot
1. Create a Linux `chroot` environment.
2. Create a `gzip` compressed `tar` file of the `chroot` directory.
- ```shell
- $ cd /path/to/chroot
- $ tar -czf /path/to/pre-receive-environment.tar.gz .
+ ```shell
+ $ cd /path/to/chroot
+ $ tar -czf /path/to/pre-receive-environment.tar.gz .
```
- {% note %}
+ {% note %}
- **Замечания:**
- - Do not include leading directory paths of files within the tar archive, such as `/path/to/chroot`.
- - `/bin/sh` must exist and be executable, as the entry point into the chroot environment.
- - Unlike traditional chroots, the `dev` directory is not required by the chroot environment for pre-receive hooks.
+ **Замечания:**
+ - Do not include leading directory paths of files within the tar archive, such as `/path/to/chroot`.
+ - `/bin/sh` must exist and be executable, as the entry point into the chroot environment.
+ - Unlike traditional chroots, the `dev` directory is not required by the chroot environment for pre-receive hooks.
- {% endnote %}
+ {% endnote %}
For more information about creating a chroot environment see "[Chroot](https://wiki.debian.org/chroot)" from the *Debian Wiki*, "[BasicChroot](https://help.ubuntu.com/community/BasicChroot)" from the *Ubuntu Community Help Wiki*, or "[Installing Alpine Linux in a chroot](http://wiki.alpinelinux.org/wiki/Installing_Alpine_Linux_in_a_chroot)" from the *Alpine Linux Wiki*.
@@ -89,4 +89,4 @@ For more information about creating a chroot environment see "[Chroot](https://w
```shell
admin@ghe-host:~$ ghe-hook-env-create AlpineTestEnv /home/admin/alpine-3.3.tar.gz
> Pre-receive hook environment 'AlpineTestEnv' (2) has been created.
- ```
+ ```
diff --git a/translations/ru-RU/content/admin/policies/creating-a-pre-receive-hook-script.md b/translations/ru-RU/content/admin/policies/creating-a-pre-receive-hook-script.md
index 53e423cee609..8a1cc8e8da2d 100644
--- a/translations/ru-RU/content/admin/policies/creating-a-pre-receive-hook-script.md
+++ b/translations/ru-RU/content/admin/policies/creating-a-pre-receive-hook-script.md
@@ -70,19 +70,19 @@ We recommend consolidating hooks to a single repository. If the consolidated hoo
```shell
$ sudo chmod +x SCRIPT_FILE.sh
- ```
- For Windows users, ensure the scripts have execute permissions:
+ ```
+ For Windows users, ensure the scripts have execute permissions:
- ```shell
- git update-index --chmod=+x SCRIPT_FILE.sh
- ```
+ ```shell
+ git update-index --chmod=+x SCRIPT_FILE.sh
+ ```
2. Commit and push to your designated pre-receive hooks repository on the {% data variables.product.prodname_ghe_server %} instance.
```shell
$ git commit -m "YOUR COMMIT MESSAGE"
$ git push
- ```
+ ```
3. [Create the pre-receive hook](/enterprise/{{ currentVersion }}/admin/guides/developer-workflow/managing-pre-receive-hooks-on-the-github-enterprise-server-appliance/#creating-pre-receive-hooks) on the {% data variables.product.prodname_ghe_server %} instance.
@@ -93,40 +93,40 @@ You can test a pre-receive hook script locally before you create or update it on
2. Create a file called `Dockerfile.dev` containing:
- ```
- FROM gliderlabs/alpine:3.3
- RUN \
- apk add --no-cache git openssh bash && \
- ssh-keygen -A && \
- sed -i "s/#AuthorizedKeysFile/AuthorizedKeysFile/g" /etc/ssh/sshd_config && \
- adduser git -D -G root -h /home/git -s /bin/bash && \
- passwd -d git && \
- su git -c "mkdir /home/git/.ssh && \
- ssh-keygen -t ed25519 -f /home/git/.ssh/id_ed25519 -P '' && \
- mv /home/git/.ssh/id_ed25519.pub /home/git/.ssh/authorized_keys && \
- mkdir /home/git/test.git && \
- git --bare init /home/git/test.git"
-
- VOLUME ["/home/git/.ssh", "/home/git/test.git/hooks"]
- WORKDIR /home/git
-
- CMD ["/usr/sbin/sshd", "-D"]
- ```
+ ```
+ FROM gliderlabs/alpine:3.3
+ RUN \
+ apk add --no-cache git openssh bash && \
+ ssh-keygen -A && \
+ sed -i "s/#AuthorizedKeysFile/AuthorizedKeysFile/g" /etc/ssh/sshd_config && \
+ adduser git -D -G root -h /home/git -s /bin/bash && \
+ passwd -d git && \
+ su git -c "mkdir /home/git/.ssh && \
+ ssh-keygen -t ed25519 -f /home/git/.ssh/id_ed25519 -P '' && \
+ mv /home/git/.ssh/id_ed25519.pub /home/git/.ssh/authorized_keys && \
+ mkdir /home/git/test.git && \
+ git --bare init /home/git/test.git"
+
+ VOLUME ["/home/git/.ssh", "/home/git/test.git/hooks"]
+ WORKDIR /home/git
+
+ CMD ["/usr/sbin/sshd", "-D"]
+ ```
3. Create a test pre-receive script called `always_reject.sh`. This example script will reject all pushes, which is useful for locking a repository:
- ```
- #!/usr/bin/env bash
+ ```
+ #!/usr/bin/env bash
- echo "error: rejecting all pushes"
- exit 1
- ```
+ echo "error: rejecting all pushes"
+ exit 1
+ ```
4. Ensure the `always_reject.sh` scripts has execute permissions:
```shell
$ chmod +x always_reject.sh
- ```
+ ```
5. From the directory containing `Dockerfile.dev`, build an image:
@@ -149,32 +149,32 @@ You can test a pre-receive hook script locally before you create or update it on
....truncated output....
> Initialized empty Git repository in /home/git/test.git/
> Successfully built dd8610c24f82
- ```
+ ```
6. Run a data container that contains a generated SSH key:
```shell
$ docker run --name data pre-receive.dev /bin/true
- ```
+ ```
7. Copy the test pre-receive hook `always_reject.sh` into the data container:
```shell
$ docker cp always_reject.sh data:/home/git/test.git/hooks/pre-receive
- ```
+ ```
8. Run an application container that runs `sshd` and executes the hook. Take note of the container id that is returned:
```shell
$ docker run -d -p 52311:22 --volumes-from data pre-receive.dev
> 7f888bc700b8d23405dbcaf039e6c71d486793cad7d8ae4dd184f4a47000bc58
- ```
+ ```
9. Copy the generated SSH key from the data container to the local machine:
```shell
$ docker cp data:/home/git/.ssh/id_ed25519 .
- ```
+ ```
10. Modify the remote of a test repository and push to the `test.git` repo within the Docker container. This example uses `git@github.com:octocat/Hello-World.git` but you can use any repo you want. This example assumes your local machine (127.0.0.1) is binding port 52311, but you can use a different IP address if docker is running on a remote machine.
@@ -193,9 +193,9 @@ You can test a pre-receive hook script locally before you create or update it on
> To git@192.168.99.100:test.git
> ! [remote rejected] main -> main (pre-receive hook declined)
> error: failed to push some refs to 'git@192.168.99.100:test.git'
- ```
+ ```
- Notice that the push was rejected after executing the pre-receive hook and echoing the output from the script.
+ Notice that the push was rejected after executing the pre-receive hook and echoing the output from the script.
### Дополнительная литература
- "[Customizing Git - An Example Git-Enforced Policy](https://git-scm.com/book/en/v2/Customizing-Git-An-Example-Git-Enforced-Policy)" from the *Pro Git website*
diff --git a/translations/ru-RU/content/admin/policies/enforcing-repository-management-policies-in-your-enterprise.md b/translations/ru-RU/content/admin/policies/enforcing-repository-management-policies-in-your-enterprise.md
index b1ad1c38b988..9fed38be0771 100644
--- a/translations/ru-RU/content/admin/policies/enforcing-repository-management-policies-in-your-enterprise.md
+++ b/translations/ru-RU/content/admin/policies/enforcing-repository-management-policies-in-your-enterprise.md
@@ -34,7 +34,7 @@ versions:
Each time someone creates a new repository on your enterprise, that person must choose a visibility for the repository. When you configure a default visibility setting for the enterprise, you choose which visibility is selected by default. For more information on repository visibility, see "[About repository visibility](/github/creating-cloning-and-archiving-repositories/about-repository-visibility)."
-If a site administrator disallows members from creating certain types of repositories, members will not be able to create that type of repository even if the visibility setting defaults to that type. For more information, see "[Setting a policy for repository creation](#setting-a-policy-for-repository-creation)."
+If an enterprise owner disallows members from creating certain types of repositories, members will not be able to create that type of repository even if the visibility setting defaults to that type. For more information, see "[Setting a policy for repository creation](#setting-a-policy-for-repository-creation)."
{% data reusables.enterprise-accounts.access-enterprise %}
{% if currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
@@ -49,9 +49,9 @@ If a site administrator disallows members from creating certain types of reposit
### Setting a policy for changing a repository's visibility
-When you prevent members from changing repository visibility, only site administrators have the ability to make public repositories private or make private repositories public.
+When you prevent members from changing repository visibility, only enterprise owners can change the visibility of a repository.
-If a site administrator has restricted repository creation to organization owners only, then members will not be able to change repository visibility. If a site administrator has restricted member repository creation to private repositories only, then members will only be able to change repositories from public to private. For more information, see "[Setting a policy for repository creation](#setting-a-policy-for-repository-creation)."
+If an enterprise owner has restricted repository creation to organization owners only, then members will not be able to change repository visibility. If an enterprise owner has restricted member repository creation to private repositories only, then members will only be able to change the visibility of a repository to private. For more information, see "[Setting a policy for repository creation](#setting-a-policy-for-repository-creation)."
{% data reusables.enterprise-accounts.access-enterprise %}
{% data reusables.enterprise-accounts.policies-tab %}
@@ -75,6 +75,15 @@ If a site administrator has restricted repository creation to organization owner
6. Under "Repository creation", use the drop-down menu and choose a policy. 
{% endif %}
+### Enforcing a policy on forking private or internal repositories
+
+Across all organizations owned by your enterprise, you can allow people with access to a private or internal repository to fork the repository, never allow forking of private or internal repositories, or allow owners to administer the setting on the organization level.
+
+{% data reusables.enterprise-accounts.access-enterprise %}
+{% data reusables.enterprise-accounts.policies-tab %}
+3. On the **Repository policies** tab, under "Repository forking", review the information about changing the setting. {% data reusables.enterprise-accounts.view-current-policy-config-orgs %}
+4. Under "Repository forking", use the drop-down menu and choose a policy. 
+
### Setting a policy for repository deletion and transfer
{% data reusables.enterprise-accounts.access-enterprise %}
@@ -166,6 +175,8 @@ You can override the default inherited settings by configuring the settings for
- **Block to the default branch** to only block force pushes to the default branch. 
6. Optionally, select **Enforce on all repositories** to override repository-specific settings. Note that this will **not** override an enterprise-wide policy. 
+{% if enterpriseServerVersions contains currentVersion %}
+
### Configuring anonymous Git read access
{% data reusables.enterprise_user_management.disclaimer-for-git-read-access %}
@@ -192,7 +203,6 @@ If necessary, you can prevent repository administrators from changing anonymous
4. Under "Anonymous Git read access", use the drop-down menu, and click **Enabled**. 
3. Optionally, to prevent repository admins from changing anonymous Git read access settings in all repositories on your enterprise, select **Prevent repository admins from changing anonymous Git read access**. 
-{% if enterpriseServerVersions contains currentVersion %}
#### Setting anonymous Git read access for a specific repository
{% data reusables.enterprise_site_admin_settings.access-settings %}
@@ -203,6 +213,7 @@ If necessary, you can prevent repository administrators from changing anonymous
6. Under "Danger Zone", next to "Enable Anonymous Git read access", click **Enable**. 
7. Review the changes. To confirm, click **Yes, enable anonymous Git read access.** 
8. Optionally, to prevent repository admins from changing this setting for this repository, select **Prevent repository admins from changing anonymous Git read access**. 
+
{% endif %}
{% if currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
diff --git a/translations/ru-RU/content/admin/release-notes.md b/translations/ru-RU/content/admin/release-notes.md
new file mode 100644
index 000000000000..d312be458ea8
--- /dev/null
+++ b/translations/ru-RU/content/admin/release-notes.md
@@ -0,0 +1,8 @@
+---
+title: Release notes
+intro: The release notes for {{ allVersions[currentVersion].versionTitle }}.
+layout: release-notes
+versions:
+ enterprise-server: '*'
+---
+
diff --git a/translations/ru-RU/content/admin/user-management/audited-actions.md b/translations/ru-RU/content/admin/user-management/audited-actions.md
index f49da01c6208..e4c495174c61 100644
--- a/translations/ru-RU/content/admin/user-management/audited-actions.md
+++ b/translations/ru-RU/content/admin/user-management/audited-actions.md
@@ -36,12 +36,12 @@ versions:
#### Enterprise configuration settings
-| Name | Description |
-| -------------------------------------------------------:| ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `business.update_member_repository_creation_permission` | A site admin restricts repository creation in organizations in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#setting-a-policy-for-repository-creation)." |
-| `business.clear_members_can_create_repos` | A site admin clears a restriction on repository creation in organizations in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#setting-a-policy-for-repository-creation)." |
-| `enterprise.config.lock_anonymous_git_access` | A site admin locks anonymous Git read access to prevent repository admins from changing existing anonymous Git read access settings for repositories in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#configuring-anonymous-git-read-access)." |
-| `enterprise.config.unlock_anonymous_git_access` | A site admin unlocks anonymous Git read access to allow repository admins to change existing anonymous Git read access settings for repositories in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#configuring-anonymous-git-read-access)." |
+| Name | Description |
+| -------------------------------------------------------:| -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `business.update_member_repository_creation_permission` | A site admin restricts repository creation in organizations in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#setting-a-policy-for-repository-creation)." |
+| `business.clear_members_can_create_repos` | A site admin clears a restriction on repository creation in organizations in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#setting-a-policy-for-repository-creation)."{% if enterpriseServerVersions contains currentVersion %}
+| `enterprise.config.lock_anonymous_git_access` | A site admin locks anonymous Git read access to prevent repository admins from changing existing anonymous Git read access settings for repositories in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#configuring-anonymous-git-read-access)." |
+| `enterprise.config.unlock_anonymous_git_access` | A site admin unlocks anonymous Git read access to allow repository admins to change existing anonymous Git read access settings for repositories in the enterprise. For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#configuring-anonymous-git-read-access)."{% endif %}
#### Issues and pull requests
@@ -77,23 +77,23 @@ versions:
#### Repositories
-| Name | Description |
-| ------------------------------------------:| -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `repo.access` | A private repository was made public, or a public repository was made private. |
-| `repo.archive` | A repository was archived. For more information, see "[Archiving a {% data variables.product.prodname_dotcom %} repository](/github/creating-cloning-and-archiving-repositories/archiving-a-github-repository)." |
-| `repo.add_member` | A collaborator was added to a repository. |
-| `repo.config` | A site admin blocked force pushes. For more information, see [Blocking force pushes to a repository](/enterprise/{{ currentVersion }}/admin/guides/developer-workflow/blocking-force-pushes-to-a-repository/) to a repository. |
-| `repo.create` | A repository was created. |
-| `repo.destroy` | A repository was deleted. |
-| `repo.remove_member` | A collaborator was removed from a repository. |
-| `repo.rename` | A repository was renamed. |
-| `repo.transfer` | A user accepted a request to receive a transferred repository. |
-| `repo.transfer_start` | A user sent a request to transfer a repository to another user or organization. |
-| `repo.unarchive` | A repository was unarchived. For more information, see "[Archiving a {% data variables.product.prodname_dotcom %} repository](/github/creating-cloning-and-archiving-repositories/archiving-a-github-repository)." |
-| `repo.config.disable_anonymous_git_access` | Anonymous Git read access is disabled for a public repository. For more information, see "[Enabling anonymous Git read access for a repository](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)." |
-| `repo.config.enable_anonymous_git_access` | Anonymous Git read access is enabled for a public repository. For more information, see "[Enabling anonymous Git read access for a repository](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)." |
-| `repo.config.lock_anonymous_git_access` | A repository's anonymous Git read access setting is locked, preventing repository administrators from changing (enabling or disabling) this setting. For more information, see "[Preventing users from changing anonymous Git read access](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)." |
-| `repo.config.unlock_anonymous_git_access` | A repository's anonymous Git read access setting is unlocked, allowing repository administrators to change (enable or disable) this setting. For more information, see "[Preventing users from changing anonymous Git read access](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)." |
+| Name | Description |
+| ------------------------------------------:| ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `repo.access` | The visibility of a repository changed to private{% if enterpriseServerVersions contains currentVersion %}, public,{% endif %} or internal. |
+| `repo.archive` | A repository was archived. For more information, see "[Archiving a {% data variables.product.prodname_dotcom %} repository](/github/creating-cloning-and-archiving-repositories/archiving-a-github-repository)." |
+| `repo.add_member` | A collaborator was added to a repository. |
+| `repo.config` | A site admin blocked force pushes. For more information, see [Blocking force pushes to a repository](/enterprise/{{ currentVersion }}/admin/guides/developer-workflow/blocking-force-pushes-to-a-repository/) to a repository. |
+| `repo.create` | A repository was created. |
+| `repo.destroy` | A repository was deleted. |
+| `repo.remove_member` | A collaborator was removed from a repository. |
+| `repo.rename` | A repository was renamed. |
+| `repo.transfer` | A user accepted a request to receive a transferred repository. |
+| `repo.transfer_start` | A user sent a request to transfer a repository to another user or organization. |
+| `repo.unarchive` | A repository was unarchived. For more information, see "[Archiving a {% data variables.product.prodname_dotcom %} repository](/github/creating-cloning-and-archiving-repositories/archiving-a-github-repository)."{% if enterpriseServerVersions contains currentVersion %}
+| `repo.config.disable_anonymous_git_access` | Anonymous Git read access is disabled for a repository. For more information, see "[Enabling anonymous Git read access for a repository](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)." |
+| `repo.config.enable_anonymous_git_access` | Anonymous Git read access is enabled for a repository. For more information, see "[Enabling anonymous Git read access for a repository](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)." |
+| `repo.config.lock_anonymous_git_access` | A repository's anonymous Git read access setting is locked, preventing repository administrators from changing (enabling or disabling) this setting. For more information, see "[Preventing users from changing anonymous Git read access](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)." |
+| `repo.config.unlock_anonymous_git_access` | A repository's anonymous Git read access setting is unlocked, allowing repository administrators to change (enable or disable) this setting. For more information, see "[Preventing users from changing anonymous Git read access](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)."{% endif %}
#### Site admin tools
diff --git a/translations/ru-RU/content/admin/user-management/managing-organizations-in-your-enterprise.md b/translations/ru-RU/content/admin/user-management/managing-organizations-in-your-enterprise.md
index 46c6cec1ac63..0bccc9095bd8 100644
--- a/translations/ru-RU/content/admin/user-management/managing-organizations-in-your-enterprise.md
+++ b/translations/ru-RU/content/admin/user-management/managing-organizations-in-your-enterprise.md
@@ -5,7 +5,7 @@ redirect_from:
- /enterprise/admin/categories/admin-bootcamp/
- /enterprise/admin/user-management/organizations-and-teams
- /enterprise/admin/user-management/managing-organizations-in-your-enterprise
-intro: 'Organizations are great for creating distinct groups of users within your company, such as divisions or groups working on similar projects. Public repositories that belong to an organization are accessible to users in other organizations, while private repositories are inaccessible to anyone but members of the organization.'
+intro: 'Organizations are great for creating distinct groups of users within your company, such as divisions or groups working on similar projects. {% if currentVersion == "github-ae@latest" %}Internal{% else %}Public and internal{% endif %} repositories that belong to an organization are accessible to users in other organizations, while private repositories are inaccessible to anyone but members of the organization that are granted access.'
mapTopic: true
versions:
enterprise-server: '*'
diff --git a/translations/ru-RU/content/developers/apps/authorizing-oauth-apps.md b/translations/ru-RU/content/developers/apps/authorizing-oauth-apps.md
index ee178121cbdb..3ef6be158c67 100644
--- a/translations/ru-RU/content/developers/apps/authorizing-oauth-apps.md
+++ b/translations/ru-RU/content/developers/apps/authorizing-oauth-apps.md
@@ -114,11 +114,13 @@ curl -H "Authorization: token OAUTH-TOKEN" {% data variables.product.api_url_pre
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
### Device flow
+{% if currentVersion ver_lt "enterprise-server@3.1" %}
{% note %}
-**Note:** The device flow is in public beta and subject to change.{% if currentVersion == "free-pro-team@latest" %} To enable this beta feature, see "[Activating beta features for apps](/developers/apps/activating-beta-features-for-apps)."{% endif %}
+**Note:** The device flow is in public beta and subject to change.
{% endnote %}
+{% endif %}
The device flow allows you to authorize users for a headless app, such as a CLI tool or Git credential manager.
diff --git a/translations/ru-RU/content/developers/apps/identifying-and-authorizing-users-for-github-apps.md b/translations/ru-RU/content/developers/apps/identifying-and-authorizing-users-for-github-apps.md
index ba6bdd62a332..f24f47be9006 100644
--- a/translations/ru-RU/content/developers/apps/identifying-and-authorizing-users-for-github-apps.md
+++ b/translations/ru-RU/content/developers/apps/identifying-and-authorizing-users-for-github-apps.md
@@ -123,11 +123,13 @@ curl -H "Authorization: token OAUTH-TOKEN" {% data variables.product.api_url_pre
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
### Device flow
+{% if currentVersion ver_lt "enterprise-server@3.1" %}
{% note %}
-**Note:** The device flow is in public beta and subject to change.{% if currentVersion == "free-pro-team@latest" %} To enable this beta feature, see "[Activating beta features for apps](/developers/apps/activating-beta-features-for-apps)."{% endif %}
+**Note:** The device flow is in public beta and subject to change.
{% endnote %}
+{% endif %}
The device flow allows you to authorize users for a headless app, such as a CLI tool or Git credential manager.
diff --git a/translations/ru-RU/content/developers/webhooks-and-events/webhook-events-and-payloads.md b/translations/ru-RU/content/developers/webhooks-and-events/webhook-events-and-payloads.md
index 2078b671233a..8eb8622fc29e 100644
--- a/translations/ru-RU/content/developers/webhooks-and-events/webhook-events-and-payloads.md
+++ b/translations/ru-RU/content/developers/webhooks-and-events/webhook-events-and-payloads.md
@@ -1288,7 +1288,7 @@ The event’s actor is the [user](/v3/users/) who starred a repository, and the
{{ webhookPayloadsForCurrentVersion.watch.started }}
-{% if currentVersion == "free-pro-team@latest" %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
### workflow_dispatch
This event occurs when someone triggers a workflow run on GitHub or sends a `POST` request to the "[Create a workflow dispatch event](/rest/reference/actions/#create-a-workflow-dispatch-event)" endpoint. For more information, see "[Events that trigger workflows](/actions/reference/events-that-trigger-workflows#workflow_dispatch)."
@@ -1302,6 +1302,7 @@ This event occurs when someone triggers a workflow run on GitHub or sends a `POS
{{ webhookPayloadsForCurrentVersion.workflow_dispatch }}
{% endif %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %}
### workflow_run
When a {% data variables.product.prodname_actions %} workflow run is requested or completed. For more information, see "[Events that trigger workflows](/actions/reference/events-that-trigger-workflows#workflow_run)."
@@ -1322,3 +1323,4 @@ When a {% data variables.product.prodname_actions %} workflow run is requested o
#### Webhook payload example
{{ webhookPayloadsForCurrentVersion.workflow_run }}
+{% endif %}
diff --git a/translations/ru-RU/content/github/administering-a-repository/about-secret-scanning.md b/translations/ru-RU/content/github/administering-a-repository/about-secret-scanning.md
index be6962f09392..25fb6899c0a7 100644
--- a/translations/ru-RU/content/github/administering-a-repository/about-secret-scanning.md
+++ b/translations/ru-RU/content/github/administering-a-repository/about-secret-scanning.md
@@ -18,6 +18,8 @@ Service providers can partner with {% data variables.product.company_short %} to
### About {% data variables.product.prodname_secret_scanning %} for public repositories
+ {% data variables.product.prodname_secret_scanning_caps %} is automatically enabled on public repositories, where it scans code for secrets, to check for known secret formats. When a match of your secret format is found in a public repository, {% data variables.product.company_short %} doesn't publicly disclose the information as an alert, but instead sends a payload to an HTTP endpoint of your choice. For an overview of how secret scanning works on public repositories, see "[Secret scanning](/developers/overview/secret-scanning)."
+
When you push to a public repository, {% data variables.product.product_name %} scans the content of the commits for secrets. If you switch a private repository to public, {% data variables.product.product_name %} scans the entire repository for secrets.
When {% data variables.product.prodname_secret_scanning %} detects a set of credentials, we notify the service provider who issued the secret. The service provider validates the credential and then decides whether they should revoke the secret, issue a new secret, or reach out to you directly, which will depend on the associated risks to you or the service provider.
@@ -65,6 +67,8 @@ When {% data variables.product.prodname_secret_scanning %} detects a set of cred
{% data reusables.secret-scanning.beta %}
+If you're a repository administrator or an organization owner, you can enable {% data variables.product.prodname_secret_scanning %} for private repositories that are owned by organizations. You can enable {% data variables.product.prodname_secret_scanning %} for all your repositories, or for all new repositories within your organization. {% data variables.product.prodname_secret_scanning_caps %} is not available for user account-owned private repositories. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository)" and "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)."
+
When you push commits to a private repository with {% data variables.product.prodname_secret_scanning %} enabled, {% data variables.product.product_name %} scans the contents of the commits for secrets.
When {% data variables.product.prodname_secret_scanning %} detects a secret in a private repository, {% data variables.product.prodname_dotcom %} sends alerts.
@@ -73,6 +77,8 @@ When {% data variables.product.prodname_secret_scanning %} detects a secret in a
- {% data variables.product.prodname_dotcom %} displays an alert in the repository. For more information, see "[Managing alerts from {% data variables.product.prodname_secret_scanning %}](/github/administering-a-repository/managing-alerts-from-secret-scanning)."
+Repository administrators and organization owners can grant users and team access to {% data variables.product.prodname_secret_scanning %} alerts. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-security-alerts)."
+
{% data variables.product.product_name %} currently scans private repositories for secrets issued by the following service providers.
- Adafruit
diff --git a/translations/ru-RU/content/github/administering-a-repository/classifying-your-repository-with-topics.md b/translations/ru-RU/content/github/administering-a-repository/classifying-your-repository-with-topics.md
index d4f1cf411bf6..43b1fa092c15 100644
--- a/translations/ru-RU/content/github/administering-a-repository/classifying-your-repository-with-topics.md
+++ b/translations/ru-RU/content/github/administering-a-repository/classifying-your-repository-with-topics.md
@@ -22,7 +22,7 @@ To browse the most used topics, go to https://github.com/topics/.
Repository admins can add any topics they'd like to a repository. Helpful topics to classify a repository include the repository's intended purpose, subject area, community, or language.{% if currentVersion == "free-pro-team@latest" %} Additionally, {% data variables.product.product_name %} analyzes public repository content and generates suggested topics that repository admins can accept or reject. Private repository content is not analyzed and does not receive topic suggestions.{% endif %}
-Public and private repositories can have topics, although you will only see private repositories that you have access to in topic search results.
+{% if currentVersion == "github-ae@latest" %}Internal {% else %}Public, internal, {% endif %}and private repositories can have topics, although you will only see private repositories that you have access to in topic search results.
You can search for repositories that are associated with a particular topic. For more information, see "[Searching for repositories](/articles/searching-for-repositories#search-by-topic)." You can also search for a list of topics on {% data variables.product.product_name %}. For more information, see "[Searching topics](/articles/searching-topics)."
diff --git a/translations/ru-RU/content/github/administering-a-repository/customizing-your-repositorys-social-media-preview.md b/translations/ru-RU/content/github/administering-a-repository/customizing-your-repositorys-social-media-preview.md
index 3c61f2eb0ed4..10a7cc56547a 100644
--- a/translations/ru-RU/content/github/administering-a-repository/customizing-your-repositorys-social-media-preview.md
+++ b/translations/ru-RU/content/github/administering-a-repository/customizing-your-repositorys-social-media-preview.md
@@ -11,7 +11,7 @@ versions:
Until you add an image, repository links expand to show basic information about the repository and the owner's avatar. Adding an image to your repository can help identify your project across various social platforms.
-You can upload an image to a private repository, but your image can only be shared from a public repository.
+{% if currentVersion != "github-ae@latest" %}You can upload an image to a private repository, but your image can only be shared from a public repository.{% endif %}
{% tip %}
Tip: Your image should be a PNG, JPG, or GIF file under 1 MB in size. For the best quality rendering, we recommend keeping the image at 640 by 320 pixels.
diff --git a/translations/ru-RU/content/github/administering-a-repository/deleting-a-repository.md b/translations/ru-RU/content/github/administering-a-repository/deleting-a-repository.md
index 95bf02446acb..df9e987dc69a 100644
--- a/translations/ru-RU/content/github/administering-a-repository/deleting-a-repository.md
+++ b/translations/ru-RU/content/github/administering-a-repository/deleting-a-repository.md
@@ -13,17 +13,16 @@ versions:
{% data reusables.organizations.owners-and-admins-can %} delete an organization repository. If **Allow members to delete or transfer repositories for this organization** has been disabled, only organization owners can delete organization repositories. {% data reusables.organizations.new-repo-permissions-more-info %}
-{% if currentVersion == "free-pro-team@latest" %}
+{% if currentVersion != "github-ae@latest" %}Deleting a public repository will not delete any forks of the repository.{% endif %}
+
{% warning %}
-**Warning**: Deleting a repository will **permanently** delete release attachments and team permissions. This action **cannot** be undone.
+**Warnings**:
-{% endwarning %}
-{% endif %}
+- Deleting a repository will **permanently** delete release attachments and team permissions. This action **cannot** be undone.
+- Deleting a private {% if currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" %}or internal {% endif %}repository will delete all forks of the repository.
-Please also keep in mind that:
-- Deleting a private repository will delete all of its forks.
-- Deleting a public repository will not delete its forks.
+{% endwarning %}
{% if currentVersion == "free-pro-team@latest" %}
You can restore some deleted repositories within 90 days. For more information, see "[Restoring a deleted repository](/articles/restoring-a-deleted-repository)."
diff --git a/translations/ru-RU/content/github/administering-a-repository/enabling-anonymous-git-read-access-for-a-repository.md b/translations/ru-RU/content/github/administering-a-repository/enabling-anonymous-git-read-access-for-a-repository.md
index 57550fe89a8b..5c740bfa0138 100644
--- a/translations/ru-RU/content/github/administering-a-repository/enabling-anonymous-git-read-access-for-a-repository.md
+++ b/translations/ru-RU/content/github/administering-a-repository/enabling-anonymous-git-read-access-for-a-repository.md
@@ -5,7 +5,6 @@ redirect_from:
- /articles/enabling-anonymous-git-read-access-for-a-repository
versions:
enterprise-server: '*'
- github-ae: '*'
---
Repository administrators can change the anonymous Git read access setting for a specific repository if:
diff --git a/translations/ru-RU/content/github/administering-a-repository/managing-repository-settings.md b/translations/ru-RU/content/github/administering-a-repository/managing-repository-settings.md
index 240e40b6ede2..fe7f46cdc3ec 100644
--- a/translations/ru-RU/content/github/administering-a-repository/managing-repository-settings.md
+++ b/translations/ru-RU/content/github/administering-a-repository/managing-repository-settings.md
@@ -1,6 +1,6 @@
---
title: Managing repository settings
-intro: 'Repository administrators and organization owners can change several settings, including the names and ownership of a repository and the public or private visibility of a repository. They can also delete a repository.'
+intro: 'Repository administrators and organization owners can change settings for a repository, like the name, ownership, and visibility, or delete the repository.'
mapTopic: true
redirect_from:
- /articles/managing-repository-settings
diff --git a/translations/ru-RU/content/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository.md b/translations/ru-RU/content/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository.md
index be534644617f..c8d4a41ef911 100644
--- a/translations/ru-RU/content/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository.md
+++ b/translations/ru-RU/content/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository.md
@@ -22,28 +22,28 @@ versions:
{% data reusables.repositories.navigate-to-security-and-analysis %}
4. Under "Configure security and analysis features", to the right of the feature, click **Disable** or **Enable**. 
-### Granting access to {% data variables.product.prodname_dependabot_alerts %}
+### Granting access to security alerts
-After you enable {% data variables.product.prodname_dependabot_alerts %} for a repository in an organization, organization owners and repository administrators can view the alerts by default. You can give additional teams and people access to the alerts for a repository.
+After you enable {% data variables.product.prodname_dependabot %} or {% data variables.product.prodname_secret_scanning %} alerts for a repository in an organization, organization owners and repository administrators can view the alerts by default. You can give additional teams and people access to the alerts for a repository.
{% note %}
-Organization owners and repository administrators can only grant access to view {% data variables.product.prodname_dependabot_alerts %} to people or teams who have write access to the repo.
+Organization owners and repository administrators can only grant access to view security alerts, such as {% data variables.product.prodname_dependabot %} and {% data variables.product.prodname_secret_scanning %} alerts, to people or teams who have write access to the repo.
{% endnote %}
{% data reusables.repositories.navigate-to-repo %}
{% data reusables.repositories.sidebar-settings %}
{% data reusables.repositories.navigate-to-security-and-analysis %}
-4. Under "Dependabot alerts", in the search field, start typing the name of the person or team you'd like to find, then click a name in the list of matches. 
-5. Click **Save changes**. 
+4. Under "Access to alerts", in the search field, start typing the name of the person or team you'd like to find, then click a name in the list of matches. 
+5. Click **Save changes**. 
-### Removing access to {% data variables.product.prodname_dependabot_alerts %}
+### Removing access to security alerts
{% data reusables.repositories.navigate-to-repo %}
{% data reusables.repositories.sidebar-settings %}
{% data reusables.repositories.navigate-to-security-and-analysis %}
-4. Under "Dependabot alerts", to the right of the person or team whose access you'd like to remove, click {% octicon "x" aria-label="X symbol" %}. 
+4. Under "Access to alerts", to the right of the person or team whose access you'd like to remove, click {% octicon "x" aria-label="X symbol" %}. 
### Дополнительная литература
diff --git a/translations/ru-RU/content/github/administering-a-repository/setting-repository-visibility.md b/translations/ru-RU/content/github/administering-a-repository/setting-repository-visibility.md
index 89a7dbadce22..2a526a1b1270 100644
--- a/translations/ru-RU/content/github/administering-a-repository/setting-repository-visibility.md
+++ b/translations/ru-RU/content/github/administering-a-repository/setting-repository-visibility.md
@@ -19,17 +19,36 @@ Organization owners can restrict the ability to change repository visibility to
We recommend reviewing the following caveats before you change the visibility of a repository.
#### Making a repository private
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+* {% data variables.product.product_name %} will detach public forks of the public repository and put them into a new network. Public forks are not made private.{% endif %}
+* If you change a repository's visibility from internal to private, {% data variables.product.prodname_dotcom %} will remove forks that belong to any user without access to the newly private repository. {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}The visibility of any forks will also change to private.{% elsif currentVersion == "github-ae@latest" %}If the internal repository has any forks, the visibility of the forks is already private.{% endif %} For more information, see "[What happens to forks when a repository is deleted or changes visibility?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility)"{% if currentVersion == "free-pro-team@latest" %}
+* If you're using {% data variables.product.prodname_free_user %} for user accounts or organizations, some features won't be available in the repository after you change the visibility to private. {% data reusables.gated-features.more-info %}{% endif %}
+* Any published {% data variables.product.prodname_pages %} site will be automatically unpublished.{% if currentVersion == "free-pro-team@latest" %} If you added a custom domain to the {% data variables.product.prodname_pages %} site, you should remove or update your DNS records before making the repository private, to avoid the risk of a domain takeover. For more information, see "[Managing a custom domain for your {% data variables.product.prodname_pages %} site](/articles/managing-a-custom-domain-for-your-github-pages-site)."{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+* {% data variables.product.prodname_dotcom %} will no longer included the repository in the {% data variables.product.prodname_archive %}. For more information, see "[About archiving content and data on {% data variables.product.prodname_dotcom %}](/github/creating-cloning-and-archiving-repositories/about-archiving-content-and-data-on-github#about-the-github-archive-program)."{% endif %}{% if enterpriseServerVersions contains currentVersion %}
+* Anonymous Git read access is no longer available. For more information, see "[Enabling anonymous Git read access for a repository](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)."{% endif %}
- * {% data variables.product.prodname_dotcom %} will detach public forks of the public repository and put them into a new network. Public forks are not made private. {% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %}If you change a repository's visibility from internal to private, {% data variables.product.prodname_dotcom %} will remove forks that belong to any user without access to the newly private repository.{% endif %} For more information, see "[What happens to forks when a repository is deleted or changes visibility?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility#changing-a-public-repository-to-a-private-repository)"
- {% if currentVersion == "free-pro-team@latest" %}* If you're using {% data variables.product.prodname_free_user %} for user accounts or organizations, some features won't be available in the repository after you change the visibility to private. {% data reusables.gated-features.more-info %}
- * Any published {% data variables.product.prodname_pages %} site will be automatically unpublished. If you added a custom domain to the {% data variables.product.prodname_pages %} site, you should remove or update your DNS records before making the repository private, to avoid the risk of a domain takeover. For more information, see "[Managing a custom domain for your {% data variables.product.prodname_pages %} site](/articles/managing-a-custom-domain-for-your-github-pages-site)."
- * {% data variables.product.prodname_dotcom %} will no longer included the repository in the {% data variables.product.prodname_archive %}. For more information, see "[About archiving content and data on {% data variables.product.prodname_dotcom %}](/github/creating-cloning-and-archiving-repositories/about-archiving-content-and-data-on-github#about-the-github-archive-program)."{% endif %}
- {% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}* Anonymous Git read access is no longer available. For more information, see "[Enabling anonymous Git read access for a repository](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)."{% endif %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %}
+
+#### Making a repository internal
+
+{% note %}
+
+**Note:** {% data reusables.gated-features.internal-repos %}
+
+{% endnote %}
+
+* Any forks of the repository will remain in the repository network, and {% data variables.product.product_name %} maintains the relationship between the root repository and the fork. For more information, see "[What happens to forks when a repository is deleted or changes visibility?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility)"
+
+{% endif %}
+
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
#### Making a repository public
- * {% data variables.product.prodname_dotcom %} will detach private forks and turn them into a standalone private repository. For more information, see "[What happens to forks when a repository is deleted or changes visibility?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility#changing-a-private-repository-to-a-public-repository)"
- * If you're converting your private repository to a public repository as part of a move toward creating an open source project, see the [Open Source Guides](http://opensource.guide) for helpful tips and guidelines.{% if currentVersion == "free-pro-team@latest" %} You can also take a free course on managing an open source project with [{% data variables.product.prodname_learning %}]({% data variables.product.prodname_learning_link %}). Once your repository is public, you can also view your repository's community profile to see whether your project meets best practices for supporting contributors. For more information, see "[Viewing your community profile](/articles/viewing-your-community-profile)."{% endif %}
+* {% data variables.product.product_name %} will detach private forks and turn them into a standalone private repository. For more information, see "[What happens to forks when a repository is deleted or changes visibility?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility#changing-a-private-repository-to-a-public-repository)"{% if currentVersion == "free-pro-team@latest" %}
+* If you're converting your private repository to a public repository as part of a move toward creating an open source project, see the [Open Source Guides](http://opensource.guide) for helpful tips and guidelines. You can also take a free course on managing an open source project with [{% data variables.product.prodname_learning %}]({% data variables.product.prodname_learning_link %}). Once your repository is public, you can also view your repository's community profile to see whether your project meets best practices for supporting contributors. For more information, see "[Viewing your community profile](/articles/viewing-your-community-profile)."{% endif %}
+
+{% endif %}
{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
diff --git a/translations/ru-RU/content/github/authenticating-to-github/about-anonymized-image-urls.md b/translations/ru-RU/content/github/authenticating-to-github/about-anonymized-image-urls.md
index 8171d9c6cd19..58d42d158e32 100644
--- a/translations/ru-RU/content/github/authenticating-to-github/about-anonymized-image-urls.md
+++ b/translations/ru-RU/content/github/authenticating-to-github/about-anonymized-image-urls.md
@@ -8,7 +8,7 @@ versions:
free-pro-team: '*'
---
-To host your images, {% data variables.product.product_name %} uses the [open-source project Camo](https://github.com/atmos/camo). Camo generates an anonymous URL proxy for each image that starts with `https://camo.githubusercontent.com/` and hides your browser details and related information from other users.
+To host your images, {% data variables.product.product_name %} uses the [open-source project Camo](https://github.com/atmos/camo). Camo generates an anonymous URL proxy for each image which hides your browser details and related information from other users. The URL starts `https://.githubusercontent.com/`, with different subdomains depending on how you uploaded the image.
Anyone who receives your anonymized image URL, directly or indirectly, may view your image. To keep sensitive images private, restrict them to a private network or a server that requires authentication instead of using Camo.
diff --git a/translations/ru-RU/content/github/authenticating-to-github/adding-a-new-ssh-key-to-your-github-account.md b/translations/ru-RU/content/github/authenticating-to-github/adding-a-new-ssh-key-to-your-github-account.md
index b13a1e250453..c3a3a069af5d 100644
--- a/translations/ru-RU/content/github/authenticating-to-github/adding-a-new-ssh-key-to-your-github-account.md
+++ b/translations/ru-RU/content/github/authenticating-to-github/adding-a-new-ssh-key-to-your-github-account.md
@@ -24,8 +24,8 @@ After adding a new SSH key to your {% data variables.product.product_name %} acc
If your SSH key file has a different name than the example code, modify the filename to match your current setup. When copying your key, don't add any newlines or whitespace.
```shell
- $ pbcopy < ~/.ssh/id_rsa.pub
- # Copies the contents of the id_rsa.pub file to your clipboard
+ $ pbcopy < ~/.ssh/id_ed25519.pub
+ # Copies the contents of the id_ed25519.pub file to your clipboard
```
{% tip %}
@@ -51,8 +51,8 @@ After adding a new SSH key to your {% data variables.product.product_name %} acc
If your SSH key file has a different name than the example code, modify the filename to match your current setup. When copying your key, don't add any newlines or whitespace.
```shell
- $ clip < ~/.ssh/id_rsa.pub
- # Copies the contents of the id_rsa.pub file to your clipboard
+ $ clip < ~/.ssh/id_ed25519.pub
+ # Copies the contents of the id_ed25519.pub file to your clipboard
```
{% tip %}
@@ -81,8 +81,8 @@ After adding a new SSH key to your {% data variables.product.product_name %} acc
$ sudo apt-get install xclip
# Downloads and installs xclip. If you don't have `apt-get`, you might need to use another installer (like `yum`)
- $ xclip -selection clipboard < ~/.ssh/id_rsa.pub
- # Copies the contents of the id_rsa.pub file to your clipboard
+ $ xclip -selection clipboard < ~/.ssh/id_ed25519.pub
+ # Copies the contents of the id_ed25519.pub file to your clipboard
```
{% tip %}
diff --git a/translations/ru-RU/content/github/authenticating-to-github/generating-a-new-ssh-key-and-adding-it-to-the-ssh-agent.md b/translations/ru-RU/content/github/authenticating-to-github/generating-a-new-ssh-key-and-adding-it-to-the-ssh-agent.md
index dc105fa0e51c..2a2c82754bf5 100644
--- a/translations/ru-RU/content/github/authenticating-to-github/generating-a-new-ssh-key-and-adding-it-to-the-ssh-agent.md
+++ b/translations/ru-RU/content/github/authenticating-to-github/generating-a-new-ssh-key-and-adding-it-to-the-ssh-agent.md
@@ -98,13 +98,19 @@ Before adding a new SSH key to the ssh-agent to manage your keys, you should hav
IdentityFile ~/.ssh/id_ed25519
```
+ {% note %}
+
+ **Note:** If you chose not to add a passphrase to your key, you should omit the `UseKeychain` line.
+
+ {% endnote %}
+
3. Add your SSH private key to the ssh-agent and store your passphrase in the keychain. {% data reusables.ssh.add-ssh-key-to-ssh-agent %}
```shell
$ ssh-add -K ~/.ssh/id_ed25519
```
{% note %}
- **Note:** The `-K` option is Apple's standard version of `ssh-add`, which stores the passphrase in your keychain for you when you add an ssh key to the ssh-agent.
+ **Note:** The `-K` option is Apple's standard version of `ssh-add`, which stores the passphrase in your keychain for you when you add an ssh key to the ssh-agent. If you chose not to add a passphrase to your key, run the command without the `-K` option.
If you don't have Apple's standard version installed, you may receive an error. For more information on resolving this error, see "[Error: ssh-add: illegal option -- K](/articles/error-ssh-add-illegal-option-k)."
diff --git a/translations/ru-RU/content/github/authenticating-to-github/reviewing-your-security-log.md b/translations/ru-RU/content/github/authenticating-to-github/reviewing-your-security-log.md
index 8d02daf16292..c38bd1da83b4 100644
--- a/translations/ru-RU/content/github/authenticating-to-github/reviewing-your-security-log.md
+++ b/translations/ru-RU/content/github/authenticating-to-github/reviewing-your-security-log.md
@@ -1,6 +1,7 @@
---
title: Reviewing your security log
intro: You can review the security log for your user account to better understand actions you've performed and actions others have performed that involve you.
+miniTocMaxHeadingLevel: 4
redirect_from:
- /articles/reviewing-your-security-log
versions:
@@ -30,216 +31,222 @@ The security log lists all actions performed within the last 90 days{% if curren
#### Search based on the action performed
{% else %}
### Understanding events in your security log
+{% endif %}
+
+The events listed in your security log are triggered by your actions. Actions are grouped into the following categories:
+
+| Category name | Description |
+| -------------------------------------------------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |{% if currentVersion == "free-pro-team@latest" %}
+| [`account_recovery_token`](#account_recovery_token-category-actions) | Contains all activities related to [adding a recovery token](/articles/configuring-two-factor-authentication-recovery-methods). |
+| [`выставление счетов`](#billing-category-actions) | Contains all activities related to your billing information. |
+| [`marketplace_agreement_signature`](#marketplace_agreement_signature-category-actions) | Contains all activities related to signing the {% data variables.product.prodname_marketplace %} Developer Agreement. |
+| [`marketplace_listing`](#marketplace_listing-category-actions) | Contains all activities related to listing apps in {% data variables.product.prodname_marketplace %}.{% endif %}
+| [`oauth_access`](#oauth_access-category-actions) | Contains all activities related to [{% data variables.product.prodname_oauth_app %}s](/articles/authorizing-oauth-apps) you've connected with.{% if currentVersion == "free-pro-team@latest" %}
+| [`payment_method`](#payment_method-category-actions) | Contains all activities related to paying for your {% data variables.product.prodname_dotcom %} subscription.{% endif %}
+| [`profile_picture`](#profile_picture-category-actions) | Contains all activities related to your profile picture. |
+| [`проект`](#project-category-actions) | Contains all activities related to project boards. |
+| [`public_key`](#public_key-category-actions) | Contains all activities related to [your public SSH keys](/articles/adding-a-new-ssh-key-to-your-github-account). |
+| [`repo`](#repo-category-actions) | Contains all activities related to the repositories you own.{% if currentVersion == "free-pro-team@latest" %}
+| [`sponsors`](#sponsors-category-actions) | Contains all events related to {% data variables.product.prodname_sponsors %} and sponsor buttons (see "[About {% data variables.product.prodname_sponsors %}](/articles/about-github-sponsors)" and "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)"){% endif %}{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
+| [`команда`](#team-category-actions) | Contains all activities related to teams you are a part of.{% endif %}{% if currentVersion != "github-ae@latest" %}
+| [`two_factor_authentication`](#two_factor_authentication-category-actions) | Contains all activities related to [two-factor authentication](/articles/securing-your-account-with-two-factor-authentication-2fa).{% endif %}
+| [`пользователь`](#user-category-actions) | Contains all activities related to your account. |
+
+{% if currentVersion == "free-pro-team@latest" %}
+
+### Exporting your security log
+
+{% data reusables.audit_log.export-log %}
+{% data reusables.audit_log.exported-log-keys-and-values %}
-Actions listed in your security log are grouped within the following categories: |{% endif %}
-| Category Name | Description |
-| ------------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |{% if currentVersion == "free-pro-team@latest" %}
-| `account_recovery_token` | Contains all activities related to [adding a recovery token](/articles/configuring-two-factor-authentication-recovery-methods). |
-| `выставление счетов` | Contains all activities related to your billing information. |
-| `marketplace_agreement_signature` | Contains all activities related to signing the {% data variables.product.prodname_marketplace %} Developer Agreement. |
-| `marketplace_listing` | Contains all activities related to listing apps in {% data variables.product.prodname_marketplace %}.{% endif %}
-| `oauth_access` | Contains all activities related to [{% data variables.product.prodname_oauth_app %}s](/articles/authorizing-oauth-apps) you've connected with.{% if currentVersion == "free-pro-team@latest" %}
-| `payment_method` | Contains all activities related to paying for your {% data variables.product.prodname_dotcom %} subscription.{% endif %}
-| `profile_picture` | Contains all activities related to your profile picture. |
-| `проект` | Contains all activities related to project boards. |
-| `public_key` | Contains all activities related to [your public SSH keys](/articles/adding-a-new-ssh-key-to-your-github-account). |
-| `repo` | Contains all activities related to the repositories you own.{% if currentVersion == "free-pro-team@latest" %}
-| `sponsors` | Contains all events related to {% data variables.product.prodname_sponsors %} and sponsor buttons (see "[About {% data variables.product.prodname_sponsors %}](/articles/about-github-sponsors)" and "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)"){% endif %}{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-| `команда` | Contains all activities related to teams you are a part of.{% endif %}{% if currentVersion != "github-ae@latest" %}
-| `two_factor_authentication` | Contains all activities related to [two-factor authentication](/articles/securing-your-account-with-two-factor-authentication-2fa).{% endif %}
-| `пользователь` | Contains all activities related to your account. |
-
-A description of the events within these categories is listed below.
+{% endif %}
+
+### Security log actions
+
+An overview of some of the most common actions that are recorded as events in the security log.
{% if currentVersion == "free-pro-team@latest" %}
-#### The `account_recovery_token` category
+#### `account_recovery_token` category actions
-| Действие | Description |
-| ------------- | ----------------------------------------------------------------------------------------------------------------------------------------------- |
-| confirm | Triggered when you successfully [store a new token with a recovery provider](/articles/configuring-two-factor-authentication-recovery-methods). |
-| recover | Triggered when you successfully [redeem an account recovery token](/articles/recovering-your-account-if-you-lose-your-2fa-credentials). |
-| recover_error | Triggered when a token is used but {% data variables.product.prodname_dotcom %} is not able to validate it. |
+| Действие | Description |
+| --------------- | ----------------------------------------------------------------------------------------------------------------------------------------------- |
+| `confirm` | Triggered when you successfully [store a new token with a recovery provider](/articles/configuring-two-factor-authentication-recovery-methods). |
+| `recover` | Triggered when you successfully [redeem an account recovery token](/articles/recovering-your-account-if-you-lose-your-2fa-credentials). |
+| `recover_error` | Triggered when a token is used but {% data variables.product.prodname_dotcom %} is not able to validate it. |
-#### The `billing` category
+#### `billing` category actions
| Действие | Description |
| --------------------- | --------------------------------------------------------------------------------------------------------------------------------------- |
-| change_billing_type | Triggered when you [change how you pay](/articles/adding-or-editing-a-payment-method) for {% data variables.product.prodname_dotcom %}. |
-| change_email | Triggered when you [change your email address](/articles/changing-your-primary-email-address). |
+| `change_billing_type` | Triggered when you [change how you pay](/articles/adding-or-editing-a-payment-method) for {% data variables.product.prodname_dotcom %}. |
+| `change_email` | Triggered when you [change your email address](/articles/changing-your-primary-email-address). |
-#### The `marketplace_agreement_signature` category
+#### `marketplace_agreement_signature` category actions
| Действие | Description |
| -------- | -------------------------------------------------------------------------------------------------- |
-| create | Triggered when you sign the {% data variables.product.prodname_marketplace %} Developer Agreement. |
+| `create` | Triggered when you sign the {% data variables.product.prodname_marketplace %} Developer Agreement. |
-#### The `marketplace_listing` category
+#### `marketplace_listing` category actions
-| Действие | Description |
-| --------- | --------------------------------------------------------------------------------------------------------------- |
-| утвердить | Triggered when your listing is approved for inclusion in {% data variables.product.prodname_marketplace %}. |
-| create | Triggered when you create a listing for your app in {% data variables.product.prodname_marketplace %}. |
-| delist | Triggered when your listing is removed from {% data variables.product.prodname_marketplace %}. |
-| redraft | Triggered when your listing is sent back to draft state. |
-| reject | Triggered when your listing is not accepted for inclusion in {% data variables.product.prodname_marketplace %}. |
+| Действие | Description |
+| ----------- | --------------------------------------------------------------------------------------------------------------- |
+| `утвердить` | Triggered when your listing is approved for inclusion in {% data variables.product.prodname_marketplace %}. |
+| `create` | Triggered when you create a listing for your app in {% data variables.product.prodname_marketplace %}. |
+| `delist` | Triggered when your listing is removed from {% data variables.product.prodname_marketplace %}. |
+| `redraft` | Triggered when your listing is sent back to draft state. |
+| `reject` | Triggered when your listing is not accepted for inclusion in {% data variables.product.prodname_marketplace %}. |
{% endif %}
-#### The `oauth_access` category
+#### `oauth_access` category actions
-| Действие | Description |
-| -------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------ |
-| create | Triggered when you [grant access to an {% data variables.product.prodname_oauth_app %}](/articles/authorizing-oauth-apps). |
-| destroy | Triggered when you [revoke an {% data variables.product.prodname_oauth_app %}'s access to your account](/articles/reviewing-your-authorized-integrations). |
+| Действие | Description |
+| --------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| `create` | Triggered when you [grant access to an {% data variables.product.prodname_oauth_app %}](/articles/authorizing-oauth-apps). |
+| `destroy` | Triggered when you [revoke an {% data variables.product.prodname_oauth_app %}'s access to your account](/articles/reviewing-your-authorized-integrations). |
{% if currentVersion == "free-pro-team@latest" %}
-#### The `payment_method` category
+#### `payment_method` category actions
-| Действие | Description |
-| ---------- | ------------------------------------------------------------------------------------------ |
-| clear | Triggered when [a payment method](/articles/removing-a-payment-method) on file is removed. |
-| create | Triggered when a new payment method is added, such as a new credit card or PayPal account. |
-| обновление | Triggered when an existing payment method is updated. |
+| Действие | Description |
+| ------------ | ------------------------------------------------------------------------------------------ |
+| `clear` | Triggered when [a payment method](/articles/removing-a-payment-method) on file is removed. |
+| `create` | Triggered when a new payment method is added, such as a new credit card or PayPal account. |
+| `обновление` | Triggered when an existing payment method is updated. |
{% endif %}
-#### The `profile_picture` category
+#### `profile_picture` category actions
-| Действие | Description |
-| ---------- | ------------------------------------------------------------------------------------------------- |
-| обновление | Triggered when you [set or update your profile picture](/articles/setting-your-profile-picture/). |
+| Действие | Description |
+| ------------ | ------------------------------------------------------------------------------------------------- |
+| `обновление` | Triggered when you [set or update your profile picture](/articles/setting-your-profile-picture/). |
-#### The `project` category
+#### `project` category actions
| Действие | Description |
| ------------------------ | ------------------------------------------------------------------------------------------------------------------------- |
+| `access` | Triggered when a project board's visibility is changed. |
| `create` | Triggered when a project board is created. |
| `rename` | Triggered when a project board is renamed. |
| `обновление` | Triggered when a project board is updated. |
| `delete - Удалить` | Triggered when a project board is deleted. |
| `link` | Triggered when a repository is linked to a project board. |
| `unlink` | Triggered when a repository is unlinked from a project board. |
-| `project.access` | Triggered when a project board's visibility is changed. |
| `update_user_permission` | Triggered when an outside collaborator is added to or removed from a project board or has their permission level changed. |
-#### The `public_key` category
+#### `public_key` category actions
-| Действие | Description |
-| ---------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| create | Triggered when you [add a new public SSH key to your {% data variables.product.product_name %} account](/articles/adding-a-new-ssh-key-to-your-github-account). |
-| delete - Удалить | Triggered when you [remove a public SSH key to your {% data variables.product.product_name %} account](/articles/reviewing-your-ssh-keys). |
+| Действие | Description |
+| ------------------ | --------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `create` | Triggered when you [add a new public SSH key to your {% data variables.product.product_name %} account](/articles/adding-a-new-ssh-key-to-your-github-account). |
+| `delete - Удалить` | Triggered when you [remove a public SSH key to your {% data variables.product.product_name %} account](/articles/reviewing-your-ssh-keys). |
-#### The `repo` category
+#### `repo` category actions
| Действие | Description |
| ------------------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| access | Triggered when you a repository you own is [switched from "private" to "public"](/articles/making-a-private-repository-public) (or vice versa). |
-| add_member | Triggered when a {% data variables.product.product_name %} user is {% if currentVersion == "free-pro-team@latest" %}[invited to have collaboration access](/articles/inviting-collaborators-to-a-personal-repository){% else %}[given collaboration access](/articles/inviting-collaborators-to-a-personal-repository){% endif %} to a repository. |
-| add_topic | Triggered when a repository owner [adds a topic](/articles/classifying-your-repository-with-topics) to a repository. |
-| archived | Triggered when a repository owner [archives a repository](/articles/about-archiving-repositories).{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-| config.disable_anonymous_git_access | Triggered when [anonymous Git read access is disabled](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository) in a public repository. |
-| config.enable_anonymous_git_access | Triggered when [anonymous Git read access is enabled](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository) in a public repository. |
-| config.lock_anonymous_git_access | Triggered when a repository's [anonymous Git read access setting is locked](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access). |
-| config.unlock_anonymous_git_access | Triggered when a repository's [anonymous Git read access setting is unlocked](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access).{% endif %}
-| create | Triggered when [a new repository is created](/articles/creating-a-new-repository). |
-| destroy | Triggered when [a repository is deleted](/articles/deleting-a-repository).{% if currentVersion == "free-pro-team@latest" %}
-| отключить | Triggered when a repository is disabled (e.g., for [insufficient funds](/articles/unlocking-a-locked-account)).{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-| включить | Triggered when a repository is re-enabled.{% endif %}
-| remove_member | Triggered when a {% data variables.product.product_name %} user is [removed from a repository as a collaborator](/articles/removing-a-collaborator-from-a-personal-repository). |
-| remove_topic | Triggered when a repository owner removes a topic from a repository. |
-| rename | Triggered when [a repository is renamed](/articles/renaming-a-repository). |
-| передача | Triggered when [a repository is transferred](/articles/how-to-transfer-a-repository). |
-| transfer_start | Triggered when a repository transfer is about to occur. |
-| unarchived | Triggered when a repository owner unarchives a repository. |
+| `access` | Triggered when you a repository you own is [switched from "private" to "public"](/articles/making-a-private-repository-public) (or vice versa). |
+| `add_member` | Triggered when a {% data variables.product.product_name %} user is {% if currentVersion == "free-pro-team@latest" %}[invited to have collaboration access](/articles/inviting-collaborators-to-a-personal-repository){% else %}[given collaboration access](/articles/inviting-collaborators-to-a-personal-repository){% endif %} to a repository. |
+| `add_topic` | Triggered when a repository owner [adds a topic](/articles/classifying-your-repository-with-topics) to a repository. |
+| `archived` | Triggered when a repository owner [archives a repository](/articles/about-archiving-repositories).{% if enterpriseServerVersions contains currentVersion %}
+| `config.disable_anonymous_git_access` | Triggered when [anonymous Git read access is disabled](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository) in a public repository. |
+| `config.enable_anonymous_git_access` | Triggered when [anonymous Git read access is enabled](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository) in a public repository. |
+| `config.lock_anonymous_git_access` | Triggered when a repository's [anonymous Git read access setting is locked](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access). |
+| `config.unlock_anonymous_git_access` | Triggered when a repository's [anonymous Git read access setting is unlocked](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access).{% endif %}
+| `create` | Triggered when [a new repository is created](/articles/creating-a-new-repository). |
+| `destroy` | Triggered when [a repository is deleted](/articles/deleting-a-repository).{% if currentVersion == "free-pro-team@latest" %}
+| `отключить` | Triggered when a repository is disabled (e.g., for [insufficient funds](/articles/unlocking-a-locked-account)).{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| `включить` | Triggered when a repository is re-enabled.{% endif %}
+| `remove_member` | Triggered when a {% data variables.product.product_name %} user is [removed from a repository as a collaborator](/articles/removing-a-collaborator-from-a-personal-repository). |
+| `remove_topic` | Triggered when a repository owner removes a topic from a repository. |
+| `rename` | Triggered when [a repository is renamed](/articles/renaming-a-repository). |
+| `передача` | Triggered when [a repository is transferred](/articles/how-to-transfer-a-repository). |
+| `transfer_start` | Triggered when a repository transfer is about to occur. |
+| `unarchived` | Triggered when a repository owner unarchives a repository. |
{% if currentVersion == "free-pro-team@latest" %}
-#### The `sponsors` category
-
-| Действие | Description |
-| ----------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
-| repo_funding_link_button_toggle | Triggered when you enable or disable a sponsor button in your repository (see "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)") |
-| repo_funding_links_file_action | Triggered when you change the FUNDING file in your repository (see "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)") |
-| sponsor_sponsorship_cancel | Triggered when you cancel a sponsorship (see "[Downgrading a sponsorship](/articles/downgrading-a-sponsorship)") |
-| sponsor_sponsorship_create | Triggered when you sponsor a developer (see "[Sponsoring an open source contributor](/github/supporting-the-open-source-community-with-github-sponsors/sponsoring-an-open-source-contributor#sponsoring-a-developer)") |
-| sponsor_sponsorship_preference_change | Triggered when you change whether you receive email updates from a sponsored developer (see "[Managing your sponsorship](/articles/managing-your-sponsorship)") |
-| sponsor_sponsorship_tier_change | Triggered when you upgrade or downgrade your sponsorship (see "[Upgrading a sponsorship](/articles/upgrading-a-sponsorship)" and "[Downgrading a sponsorship](/articles/downgrading-a-sponsorship)") |
-| sponsored_developer_approve | Triggered when your {% data variables.product.prodname_sponsors %} account is approved (see "[Setting up {% data variables.product.prodname_sponsors %} for your user account](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
-| sponsored_developer_create | Triggered when your {% data variables.product.prodname_sponsors %} account is created (see "[Setting up {% data variables.product.prodname_sponsors %} for your user account](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
-| sponsored_developer_profile_update | Triggered when you edit your sponsored developer profile (see "[Editing your profile details for {% data variables.product.prodname_sponsors %}](/github/supporting-the-open-source-community-with-github-sponsors/editing-your-profile-details-for-github-sponsors)") |
-| sponsored_developer_request_approval | Triggered when you submit your application for {% data variables.product.prodname_sponsors %} for approval (see "[Setting up {% data variables.product.prodname_sponsors %} for your user account](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
-| sponsored_developer_tier_description_update | Triggered when you change the description for a sponsorship tier (see "[Changing your sponsorship tiers](/articles/changing-your-sponsorship-tiers)") |
-| sponsored_developer_update_newsletter_send | Triggered when you send an email update to your sponsors (see "[Contacting your sponsors](/articles/contacting-your-sponsors)") |
-| waitlist_invite_sponsored_developer | Triggered when you are invited to join {% data variables.product.prodname_sponsors %} from the waitlist (see "[Setting up {% data variables.product.prodname_sponsors %} for your user account](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
-| waitlist_join | Triggered when you join the waitlist to become a sponsored developer (see "[Setting up {% data variables.product.prodname_sponsors %} for your user account](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
+#### `sponsors` category actions
+
+| Действие | Description |
+| --------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| `repo_funding_link_button_toggle` | Triggered when you enable or disable a sponsor button in your repository (see "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)") |
+| `repo_funding_links_file_action` | Triggered when you change the FUNDING file in your repository (see "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)") |
+| `sponsor_sponsorship_cancel` | Triggered when you cancel a sponsorship (see "[Downgrading a sponsorship](/articles/downgrading-a-sponsorship)") |
+| `sponsor_sponsorship_create` | Triggered when you sponsor a developer (see "[Sponsoring an open source contributor](/github/supporting-the-open-source-community-with-github-sponsors/sponsoring-an-open-source-contributor#sponsoring-a-developer)") |
+| `sponsor_sponsorship_preference_change` | Triggered when you change whether you receive email updates from a sponsored developer (see "[Managing your sponsorship](/articles/managing-your-sponsorship)") |
+| `sponsor_sponsorship_tier_change` | Triggered when you upgrade or downgrade your sponsorship (see "[Upgrading a sponsorship](/articles/upgrading-a-sponsorship)" and "[Downgrading a sponsorship](/articles/downgrading-a-sponsorship)") |
+| `sponsored_developer_approve` | Triggered when your {% data variables.product.prodname_sponsors %} account is approved (see "[Setting up {% data variables.product.prodname_sponsors %} for your user account](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
+| `sponsored_developer_create` | Triggered when your {% data variables.product.prodname_sponsors %} account is created (see "[Setting up {% data variables.product.prodname_sponsors %} for your user account](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
+| `sponsored_developer_profile_update` | Triggered when you edit your sponsored developer profile (see "[Editing your profile details for {% data variables.product.prodname_sponsors %}](/github/supporting-the-open-source-community-with-github-sponsors/editing-your-profile-details-for-github-sponsors)") |
+| `sponsored_developer_request_approval` | Triggered when you submit your application for {% data variables.product.prodname_sponsors %} for approval (see "[Setting up {% data variables.product.prodname_sponsors %} for your user account](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
+| `sponsored_developer_tier_description_update` | Triggered when you change the description for a sponsorship tier (see "[Changing your sponsorship tiers](/articles/changing-your-sponsorship-tiers)") |
+| `sponsored_developer_update_newsletter_send` | Triggered when you send an email update to your sponsors (see "[Contacting your sponsors](/articles/contacting-your-sponsors)") |
+| `waitlist_invite_sponsored_developer` | Triggered when you are invited to join {% data variables.product.prodname_sponsors %} from the waitlist (see "[Setting up {% data variables.product.prodname_sponsors %} for your user account](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
+| `waitlist_join` | Triggered when you join the waitlist to become a sponsored developer (see "[Setting up {% data variables.product.prodname_sponsors %} for your user account](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)") |
{% endif %}
{% if currentVersion == "free-pro-team@latest" %}
-#### The `successor_invitation` category
-
-| Действие | Description |
-| -------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| accept | Triggered when you accept a succession invitation (see "[Maintaining ownership continuity of your user account's repositories](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
-| cancel | Triggered when you cancel a succession invitation (see "[Maintaining ownership continuity of your user account's repositories](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
-| create | Triggered when you create a succession invitation (see "[Maintaining ownership continuity of your user account's repositories](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
-| decline | Triggered when you decline a succession invitation (see "[Maintaining ownership continuity of your user account's repositories](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
-| revoke | Triggered when you revoke a succession invitation (see "[Maintaining ownership continuity of your user account's repositories](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
+#### `successor_invitation` category actions
+
+| Действие | Description |
+| --------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `accept` | Triggered when you accept a succession invitation (see "[Maintaining ownership continuity of your user account's repositories](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
+| `cancel` | Triggered when you cancel a succession invitation (see "[Maintaining ownership continuity of your user account's repositories](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
+| `create` | Triggered when you create a succession invitation (see "[Maintaining ownership continuity of your user account's repositories](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
+| `decline` | Triggered when you decline a succession invitation (see "[Maintaining ownership continuity of your user account's repositories](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
+| `revoke` | Triggered when you revoke a succession invitation (see "[Maintaining ownership continuity of your user account's repositories](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)") |
{% endif %}
{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-#### The `team` category
+#### `team` category actions
-| Действие | Description |
-| ----------------- | --------------------------------------------------------------------------------------------------------------------------------------------- |
-| add_member | Triggered when a member of an organization you belong to [adds you to a team](/articles/adding-organization-members-to-a-team). |
-| add_repository | Triggered when a team you are a member of is given control of a repository. |
-| create | Triggered when a new team in an organization you belong to is created. |
-| destroy | Triggered when a team you are a member of is deleted from the organization. |
-| remove_member | Triggered when a member of an organization is [removed from a team](/articles/removing-organization-members-from-a-team) you are a member of. |
-| remove_repository | Triggered when a repository is no longer under a team's control. |
+| Действие | Description |
+| ------------------- | --------------------------------------------------------------------------------------------------------------------------------------------- |
+| `add_member` | Triggered when a member of an organization you belong to [adds you to a team](/articles/adding-organization-members-to-a-team). |
+| `add_repository` | Triggered when a team you are a member of is given control of a repository. |
+| `create` | Triggered when a new team in an organization you belong to is created. |
+| `destroy` | Triggered when a team you are a member of is deleted from the organization. |
+| `remove_member` | Triggered when a member of an organization is [removed from a team](/articles/removing-organization-members-from-a-team) you are a member of. |
+| `remove_repository` | Triggered when a repository is no longer under a team's control. |
{% endif %}
{% if currentVersion != "github-ae@latest" %}
-#### The `two_factor_authentication` category
+#### `two_factor_authentication` category actions
-| Действие | Description |
-| -------- | -------------------------------------------------------------------------------------------------------------------------- |
-| enabled | Triggered when [two-factor authentication](/articles/securing-your-account-with-two-factor-authentication-2fa) is enabled. |
-| disabled | Triggered when two-factor authentication is disabled. |
+| Действие | Description |
+| ---------- | -------------------------------------------------------------------------------------------------------------------------- |
+| `enabled` | Triggered when [two-factor authentication](/articles/securing-your-account-with-two-factor-authentication-2fa) is enabled. |
+| `disabled` | Triggered when two-factor authentication is disabled. |
{% endif %}
-#### The `user` category
-
-| Действие | Description |
-| ---------------------------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| add_email | Triggered when you {% if currentVersion != "github-ae@latest" %}[add a new email address](/articles/changing-your-primary-email-address){% else %}add a new email address{% endif %}. |
-| create | Triggered when you create a new user account. |
-| remove_email | Triggered when you remove an email address. |
-| rename | Triggered when you rename your account.{% if currentVersion != "github-ae@latest" %}
-| change_password | Triggered when you change your password. |
-| forgot_password | Triggered when you ask for [a password reset](/articles/how-can-i-reset-my-password).{% endif %}
-| login | Triggered when you log in to {% data variables.product.product_location %}. |
-| failed_login | Triggered when you failed to log in successfully.{% if currentVersion != "github-ae@latest" %}
-| two_factor_requested | Triggered when {% data variables.product.product_name %} asks you for [your two-factor authentication code](/articles/accessing-github-using-two-factor-authentication).{% endif %}
-| show_private_contributions_count | Triggered when you [publicize private contributions on your profile](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile). |
-| hide_private_contributions_count | Triggered when you [hide private contributions on your profile](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile).{% if currentVersion == "free-pro-team@latest" %}
-| report_content | Triggered when you [report an issue or pull request, or a comment on an issue, pull request, or commit](/articles/reporting-abuse-or-spam).{% endif %}
-
-#### The `user_status` category
-
-| Действие | Description |
-| ---------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| обновление | Triggered when you set or change the status on your profile. For more information, see "[Setting a status](/articles/personalizing-your-profile/#setting-a-status)." |
-| destroy | Triggered when you clear the status on your profile. |
+#### `user` category actions
-{% if currentVersion == "free-pro-team@latest" %}
+| Действие | Description |
+| ---------------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `add_email` | Triggered when you {% if currentVersion != "github-ae@latest" %}[add a new email address](/articles/changing-your-primary-email-address){% else %}add a new email address{% endif %}. |
+| `create` | Triggered when you create a new user account.{% if currentVersion != "github-ae@latest" %}
+| `change_password` | Triggered when you change your password. |
+| `forgot_password` | Triggered when you ask for [a password reset](/articles/how-can-i-reset-my-password).{% endif %}
+| `hide_private_contributions_count` | Triggered when you [hide private contributions on your profile](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile). |
+| `login` | Triggered when you log in to {% data variables.product.product_location %}. |
+| `failed_login` | Triggered when you failed to log in successfully. |
+| `remove_email` | Triggered when you remove an email address. |
+| `rename` | Triggered when you rename your account.{% if currentVersion == "free-pro-team@latest" %}
+| `report_content` | Triggered when you [report an issue or pull request, or a comment on an issue, pull request, or commit](/articles/reporting-abuse-or-spam).{% endif %}
+| `show_private_contributions_count` | Triggered when you [publicize private contributions on your profile](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile).{% if currentVersion != "github-ae@latest" %}
+| `two_factor_requested` | Triggered when {% data variables.product.product_name %} asks you for [your two-factor authentication code](/articles/accessing-github-using-two-factor-authentication).{% endif %}
-### Exporting your security log
+#### `user_status` category actions
+
+| Действие | Description |
+| ------------ | -------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `обновление` | Triggered when you set or change the status on your profile. For more information, see "[Setting a status](/articles/personalizing-your-profile/#setting-a-status)." |
+| `destroy` | Triggered when you clear the status on your profile. |
-{% data reusables.audit_log.export-log %}
-{% data reusables.audit_log.exported-log-keys-and-values %}
-{% endif %}
diff --git a/translations/ru-RU/content/github/building-a-strong-community/about-issue-and-pull-request-templates.md b/translations/ru-RU/content/github/building-a-strong-community/about-issue-and-pull-request-templates.md
index c45a6e764fc6..8d8c0a7b0e5b 100644
--- a/translations/ru-RU/content/github/building-a-strong-community/about-issue-and-pull-request-templates.md
+++ b/translations/ru-RU/content/github/building-a-strong-community/about-issue-and-pull-request-templates.md
@@ -11,7 +11,11 @@ versions:
After you create issue and pull request templates in your repository, contributors can use the templates to open issues or describe the proposed changes in their pull requests according to the repository's contributing guidelines. For more information about adding contributing guidelines to a repository, see "[Setting guidelines for repository contributors](/articles/setting-guidelines-for-repository-contributors)."
-You can create default issue and pull request templates for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. For more information, see "[Creating a default community health file](/github/building-a-strong-community/creating-a-default-community-health-file)."
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+You can create default issue and pull request templates for your organization or user account. For more information, see "[Creating a default community health file](/github/building-a-strong-community/creating-a-default-community-health-file)."
+
+{% endif %}
### Issue templates
diff --git a/translations/ru-RU/content/github/building-a-strong-community/about-wikis.md b/translations/ru-RU/content/github/building-a-strong-community/about-wikis.md
index 9107a139078c..8696c9c3ab4c 100644
--- a/translations/ru-RU/content/github/building-a-strong-community/about-wikis.md
+++ b/translations/ru-RU/content/github/building-a-strong-community/about-wikis.md
@@ -15,9 +15,9 @@ Every {% data variables.product.product_name %} repository comes equipped with a
With wikis, you can write content just like everywhere else on {% data variables.product.product_name %}. For more information, see "[Getting started with writing and formatting on {% data variables.product.prodname_dotcom %}](/articles/getting-started-with-writing-and-formatting-on-github)." We use [our open-source Markup library](https://github.com/github/markup) to convert different formats into HTML, so you can choose to write in Markdown or any other supported format.
-Wikis are available to the public in public repositories, and limited to people with access to the repository in private repositories. For more information, see "[Setting repository visibility](/articles/setting-repository-visibility)."
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}If you create a wiki in a public repository, the wiki is available to {% if enterpriseServerVersions contains currentVersion %}anyone with access to {% data variables.product.product_location %}{% else %}the public{% endif %}. {% endif %}If you create a wiki in an internal or private repository, {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}people{% elsif currentVersion == "github-ae@latest" %}enterprise members{% endif %} with access to the repository can also access the wiki. For more information, see "[Setting repository visibility](/articles/setting-repository-visibility)."
-You can edit wikis directly on {% data variables.product.product_name %}, or you can edit wiki files locally. By default, only people with write access to your repository can make changes to wikis, although you can allow everyone on {% data variables.product.product_name %} to contribute to a wiki in a public repository. For more information, see "[Changing access permissions for wikis](/articles/changing-access-permissions-for-wikis)".
+You can edit wikis directly on {% data variables.product.product_name %}, or you can edit wiki files locally. By default, only people with write access to your repository can make changes to wikis, although you can allow everyone on {% data variables.product.product_location %} to contribute to a wiki in {% if currentVersion == "github-ae@latest" %}an internal{% else %}a public{% endif %} repository. For more information, see "[Changing access permissions for wikis](/articles/changing-access-permissions-for-wikis)".
### Дополнительная литература
diff --git a/translations/ru-RU/content/github/building-a-strong-community/adding-a-license-to-a-repository.md b/translations/ru-RU/content/github/building-a-strong-community/adding-a-license-to-a-repository.md
index 4d0c21f0119b..6eff19a0d7f3 100644
--- a/translations/ru-RU/content/github/building-a-strong-community/adding-a-license-to-a-repository.md
+++ b/translations/ru-RU/content/github/building-a-strong-community/adding-a-license-to-a-repository.md
@@ -6,7 +6,6 @@ redirect_from:
versions:
free-pro-team: '*'
enterprise-server: '*'
- github-ae: '*'
---
If you include a detectable license in your repository, people who visit your repository will see it at the top of the repository page. To read the entire license file, click the license name.
diff --git a/translations/ru-RU/content/github/building-a-strong-community/adding-support-resources-to-your-project.md b/translations/ru-RU/content/github/building-a-strong-community/adding-support-resources-to-your-project.md
index 7856e3860fd5..397caf7fcfe8 100644
--- a/translations/ru-RU/content/github/building-a-strong-community/adding-support-resources-to-your-project.md
+++ b/translations/ru-RU/content/github/building-a-strong-community/adding-support-resources-to-your-project.md
@@ -13,7 +13,11 @@ To direct people to specific support resources, you can add a SUPPORT file to yo

-You can create default support resources for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. For more information, see "[Creating a default community health file](/github/building-a-strong-community/creating-a-default-community-health-file)."
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+You can create default support resources for your organization or user account. For more information, see "[Creating a default community health file](/github/building-a-strong-community/creating-a-default-community-health-file)."
+
+{% endif %}
{% tip %}
diff --git a/translations/ru-RU/content/github/building-a-strong-community/configuring-issue-templates-for-your-repository.md b/translations/ru-RU/content/github/building-a-strong-community/configuring-issue-templates-for-your-repository.md
index cf15d01712b9..265bcf2501d4 100644
--- a/translations/ru-RU/content/github/building-a-strong-community/configuring-issue-templates-for-your-repository.md
+++ b/translations/ru-RU/content/github/building-a-strong-community/configuring-issue-templates-for-your-repository.md
@@ -10,10 +10,16 @@ versions:
github-ae: '*'
---
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
{% data reusables.repositories.default-issue-templates %}
+{% endif %}
+
{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %}
+
### Creating issue templates
+
{% endif %}
{% data reusables.repositories.navigate-to-repo %}
diff --git a/translations/ru-RU/content/github/building-a-strong-community/creating-a-default-community-health-file.md b/translations/ru-RU/content/github/building-a-strong-community/creating-a-default-community-health-file.md
index bc1857cf9442..9cc3d4d0b3ee 100644
--- a/translations/ru-RU/content/github/building-a-strong-community/creating-a-default-community-health-file.md
+++ b/translations/ru-RU/content/github/building-a-strong-community/creating-a-default-community-health-file.md
@@ -7,7 +7,6 @@ redirect_from:
versions:
free-pro-team: '*'
enterprise-server: '*'
- github-ae: '*'
---
### About default community health files
diff --git a/translations/ru-RU/content/github/building-a-strong-community/creating-a-pull-request-template-for-your-repository.md b/translations/ru-RU/content/github/building-a-strong-community/creating-a-pull-request-template-for-your-repository.md
index 2dd9b5923f46..338dcfcd0b50 100644
--- a/translations/ru-RU/content/github/building-a-strong-community/creating-a-pull-request-template-for-your-repository.md
+++ b/translations/ru-RU/content/github/building-a-strong-community/creating-a-pull-request-template-for-your-repository.md
@@ -13,7 +13,11 @@ For more information, see "[About issue and pull request templates](/articles/ab
You can create a *PULL_REQUEST_TEMPLATE/* subdirectory in any of the supported folders to contain multiple pull request templates, and use the `template` query parameter to specify the template that will fill the pull request body. For more information, see "[About automation for issues and pull requests with query parameters](/articles/about-automation-for-issues-and-pull-requests-with-query-parameters)."
-You can create default pull request templates for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. For more information, see "[Creating a default community health file](/github/building-a-strong-community/creating-a-default-community-health-file)."
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+You can create default pull request templates for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. For more information, see "[Creating a default community health file](/github/building-a-strong-community/creating-a-default-community-health-file)."
+
+{% endif %}
### Adding a pull request template
diff --git a/translations/ru-RU/content/github/building-a-strong-community/locking-conversations.md b/translations/ru-RU/content/github/building-a-strong-community/locking-conversations.md
index 29dc92cdc754..d21cbd07924b 100644
--- a/translations/ru-RU/content/github/building-a-strong-community/locking-conversations.md
+++ b/translations/ru-RU/content/github/building-a-strong-community/locking-conversations.md
@@ -15,7 +15,7 @@ Locking a conversation creates a timeline event that is visible to anyone with r

-While a conversation is locked, only [people with write access](/articles/repository-permission-levels-for-an-organization/) and [repository owners and collaborators](/articles/permission-levels-for-a-user-account-repository/#collaborator-access-on-a-repository-owned-by-a-user-account) can add, hide, and delete comments.
+While a conversation is locked, only [people with write access](/articles/repository-permission-levels-for-an-organization/) and [repository owners and collaborators](/articles/permission-levels-for-a-user-account-repository/#collaborator-access-for-a-repository-owned-by-a-user-account) can add, hide, and delete comments.
To search for locked conversations in a repository that is not archived, you can use the search qualifiers `is:locked` and `archived:false`. Conversations are automatically locked in archived repositories. For more information, see "[Searching issues and pull requests](/articles/searching-issues-and-pull-requests#search-based-on-whether-a-conversation-is-locked)."
diff --git a/translations/ru-RU/content/github/building-a-strong-community/manually-creating-a-single-issue-template-for-your-repository.md b/translations/ru-RU/content/github/building-a-strong-community/manually-creating-a-single-issue-template-for-your-repository.md
index cfb7712c8a2c..77d6f3b744bf 100644
--- a/translations/ru-RU/content/github/building-a-strong-community/manually-creating-a-single-issue-template-for-your-repository.md
+++ b/translations/ru-RU/content/github/building-a-strong-community/manually-creating-a-single-issue-template-for-your-repository.md
@@ -39,8 +39,12 @@ assignees: octocat
{% endif %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
{% data reusables.repositories.default-issue-templates %}
+{% endif %}
+
### Adding an issue template
{% data reusables.repositories.navigate-to-repo %}
diff --git a/translations/ru-RU/content/github/building-a-strong-community/setting-guidelines-for-repository-contributors.md b/translations/ru-RU/content/github/building-a-strong-community/setting-guidelines-for-repository-contributors.md
index ddb9b5d67d26..fdbe24357601 100644
--- a/translations/ru-RU/content/github/building-a-strong-community/setting-guidelines-for-repository-contributors.md
+++ b/translations/ru-RU/content/github/building-a-strong-community/setting-guidelines-for-repository-contributors.md
@@ -20,7 +20,11 @@ For contributors, the guidelines help them verify that they're submitting well-f
For both owners and contributors, contribution guidelines save time and hassle caused by improperly created pull requests or issues that have to be rejected and re-submitted.
-You can create default contribution guidelines for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. For more information, see "[Creating a default community health file](/github/building-a-strong-community/creating-a-default-community-health-file)."
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+You can create default contribution guidelines for your organization{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" %} or user account{% endif %}. For more information, see "[Creating a default community health file](/github/building-a-strong-community/creating-a-default-community-health-file)."
+
+{% endif %}
{% tip %}
@@ -53,5 +57,5 @@ If you're stumped, here are some good examples of contribution guidelines:
### Дополнительная литература
- The Open Source Guides' section "[Starting an Open Source Project](https://opensource.guide/starting-a-project/)"{% if currentVersion == "free-pro-team@latest" %}
-- [{% data variables.product.prodname_learning %}]({% data variables.product.prodname_learning_link %}){% endif %}
-- "[Adding a license to a repository](/articles/adding-a-license-to-a-repository)"
+- [{% data variables.product.prodname_learning %}]({% data variables.product.prodname_learning_link %}){% endif %}{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- "[Adding a license to a repository](/articles/adding-a-license-to-a-repository)"{% endif %}
diff --git a/translations/ru-RU/content/github/collaborating-with-issues-and-pull-requests/about-branches.md b/translations/ru-RU/content/github/collaborating-with-issues-and-pull-requests/about-branches.md
index bc0c92b6a069..4a56f8edddfc 100644
--- a/translations/ru-RU/content/github/collaborating-with-issues-and-pull-requests/about-branches.md
+++ b/translations/ru-RU/content/github/collaborating-with-issues-and-pull-requests/about-branches.md
@@ -23,7 +23,7 @@ You must have write access to a repository to create a branch, open a pull reque
### About the default branch
-{% data reusables.branches.new-repo-default-branch %} The default branch is the branch that {% data variables.product.prodname_dotcom %} displays when anyone visits your repository. The default branch is also the initial branch that Git checks out locally out when someone clones the repository. {% data reusables.branches.default-branch-automatically-base-branch %}
+{% data reusables.branches.new-repo-default-branch %} The default branch is the branch that {% data variables.product.prodname_dotcom %} displays when anyone visits your repository. The default branch is also the initial branch that Git checks out locally when someone clones the repository. {% data reusables.branches.default-branch-automatically-base-branch %}
By default, {% data variables.product.product_name %} names the default branch {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}`main`{% else %}`master`{% endif %} in any new repository.
@@ -75,7 +75,7 @@ When a branch is protected:
- If required pull request reviews are enabled on the branch, you won't be able to merge changes into the branch until all requirements in the pull request review policy have been met. For more information, see "[Merging a pull request](/articles/merging-a-pull-request)."
- If required review from a code owner is enabled on a branch, and a pull request modifies code that has an owner, a code owner must approve the pull request before it can be merged. For more information, see "[About code owners](/articles/about-code-owners)."
- If required commit signing is enabled on a branch, you won't be able to push any commits to the branch that are not signed and verified. For more information, see "[About commit signature verification](/articles/about-commit-signature-verification)" and "[About required commit signing](/articles/about-required-commit-signing)."{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
-- If you use {% data variables.product.prodname_dotcom %} 's conflict editor to fix conflicts for a pull request that you created from a protected branch, {% data variables.product.prodname_dotcom %} helps you to create an alternative branch for the pull request, so that your resolution of the conflicts can be merged. For more information, see "[Resolving a merge conflict on {% data variables.product.prodname_dotcom %}](/github/collaborating-with-issues-and-pull-requests/resolving-a-merge-conflict-on-github)."{% endif %}
+- If you use {% data variables.product.prodname_dotcom %}'s conflict editor to fix conflicts for a pull request that you created from a protected branch, {% data variables.product.prodname_dotcom %} helps you to create an alternative branch for the pull request, so that your resolution of the conflicts can be merged. For more information, see "[Resolving a merge conflict on {% data variables.product.prodname_dotcom %}](/github/collaborating-with-issues-and-pull-requests/resolving-a-merge-conflict-on-github)."{% endif %}
### Дополнительная литература
diff --git a/translations/ru-RU/content/github/collaborating-with-issues-and-pull-requests/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility.md b/translations/ru-RU/content/github/collaborating-with-issues-and-pull-requests/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility.md
index f789d8917a1f..ac092e2c45d2 100644
--- a/translations/ru-RU/content/github/collaborating-with-issues-and-pull-requests/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility.md
+++ b/translations/ru-RU/content/github/collaborating-with-issues-and-pull-requests/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility.md
@@ -16,14 +16,20 @@ versions:
When you delete a private repository, all of its private forks are also deleted.
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
#### Deleting a public repository
When you delete a public repository, one of the existing public forks is chosen to be the new parent repository. All other repositories are forked off of this new parent and subsequent pull requests go to this new parent.
+{% endif %}
+
#### Private forks and permissions
{% data reusables.repositories.private_forks_inherit_permissions %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
#### Changing a public repository to a private repository
If a public repository is made private, its public forks are split off into a new network. As with deleting a public repository, one of the existing public forks is chosen to be the new parent repository and all other repositories are forked off of this new parent. Subsequent pull requests go to this new parent.
@@ -46,9 +52,30 @@ If a private repository is made public, each of its private forks is turned into
If a private repository is made public and then deleted, its private forks will continue to exist as standalone private repositories in separate networks.
+{% endif %}
+
+{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %}
+
+#### Changing the visibility of an internal repository
+
+{% note %}
+
+**Note:** {% data reusables.gated-features.internal-repos %}
+
+{% endnote %}
+
+If the policy for your enterprise permits forking, any fork of an internal repository will be private. If you change the visibility of an internal repository, any fork owned by an organization or user account will remain private.
+
+##### Deleting the internal repository
+
+If you change the visibility of an internal repository and then delete the repository, the forks will continue to exist in a separate network.
+
+{% endif %}
+
### Дополнительная литература
- "[Setting repository visibility](/articles/setting-repository-visibility)"
- "[About forks](/articles/about-forks)"
- "[Managing the forking policy for your repository](/github/administering-a-repository/managing-the-forking-policy-for-your-repository)"
- "[Managing the forking policy for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-the-forking-policy-for-your-organization)"
+- "{% if currentVersion == "free-pro-team@latest" %}[Enforcing repository management policies in your enterprise account](/github/setting-up-and-managing-your-enterprise/enforcing-repository-management-policies-in-your-enterprise-account#enforcing-a-policy-on-forking-private-or-internal-repositories){% else %}[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#enforcing-a-policy-on-forking-private-or-internal-repositories){% endif %}"
diff --git a/translations/ru-RU/content/github/creating-cloning-and-archiving-repositories/about-readmes.md b/translations/ru-RU/content/github/creating-cloning-and-archiving-repositories/about-readmes.md
index 3215dc181818..92a11594592d 100644
--- a/translations/ru-RU/content/github/creating-cloning-and-archiving-repositories/about-readmes.md
+++ b/translations/ru-RU/content/github/creating-cloning-and-archiving-repositories/about-readmes.md
@@ -11,7 +11,11 @@ versions:
github-ae: '*'
---
-A README file, along with {% if currentVersion == "free-pro-team@latest" %}a [repository license](/articles/licensing-a-repository), [contribution guidelines](/articles/setting-guidelines-for-repository-contributors), and a [code of conduct](/articles/adding-a-code-of-conduct-to-your-project){% else %}a [repository license](/articles/licensing-a-repository) and [contribution guidelines](/articles/setting-guidelines-for-repository-contributors){% endif %}, helps you communicate expectations for and manage contributions to your project.
+### About READMEs
+
+You can add a README file to a repository to communicate important information about your project. A README, along with a repository license{% if currentVersion == "free-pro-team@latest" %}, contribution guidelines, and a code of conduct{% elsif enterpriseServerVersions contains currentVersion %} and contribution guidelines{% endif %}, communicates expectations for your project and helps you manage contributions.
+
+For more information about providing guidelines for your project, see {% if currentVersion == "free-pro-team@latest" %}"[Adding a code of conduct to your project](/github/building-a-strong-community/adding-a-code-of-conduct-to-your-project)" and {% endif %}"[Setting up your project for healthy contributions](/github/building-a-strong-community/setting-up-your-project-for-healthy-contributions)."
A README is often the first item a visitor will see when visiting your repository. README files typically include information on:
- What the project does
@@ -26,8 +30,12 @@ If you put your README file in your repository's root, `docs`, or hidden `.githu
{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21"%}
+
{% data reusables.profile.profile-readme %}
+{% endif %}
+

{% endif %}
diff --git a/translations/ru-RU/content/github/creating-cloning-and-archiving-repositories/licensing-a-repository.md b/translations/ru-RU/content/github/creating-cloning-and-archiving-repositories/licensing-a-repository.md
index 79e5456127a8..be136a291c88 100644
--- a/translations/ru-RU/content/github/creating-cloning-and-archiving-repositories/licensing-a-repository.md
+++ b/translations/ru-RU/content/github/creating-cloning-and-archiving-repositories/licensing-a-repository.md
@@ -7,7 +7,6 @@ redirect_from:
versions:
free-pro-team: '*'
enterprise-server: '*'
- github-ae: '*'
---
### Choosing the right license
@@ -18,7 +17,7 @@ You're under no obligation to choose a license. However, without a license, the
{% note %}
-**Note:** If you publish your source code in a public repository on GitHub, {% if currentVersion == "free-pro-team@latest" %}according to the [Terms of Service](/articles/github-terms-of-service), {% endif %}other GitHub users have the right to view and fork your repository within the GitHub site. If you have already created a public repository and no longer want users to have access to it, you can make your repository private. When you convert a public repository to a private repository, existing forks or local copies created by other users will still exist. For more information, see "[Making a public repository private](/articles/making-a-public-repository-private)."
+**Note:** If you publish your source code in a public repository on {% data variables.product.product_name %}, {% if currentVersion == "free-pro-team@latest" %}according to the [Terms of Service](/articles/github-terms-of-service), {% endif %}other users of {% data variables.product.product_location %} have the right to view and fork your repository. If you have already created a repository and no longer want users to have access to the repository, you can make the repository private. When you change the visibility of a repository to private, existing forks or local copies created by other users will still exist. For more information, see "[Setting repository visibility](/github/administering-a-repository/setting-repository-visibility)."
{% endnote %}
diff --git a/translations/ru-RU/content/github/developing-online-with-codespaces/using-codespaces-in-visual-studio.md b/translations/ru-RU/content/github/developing-online-with-codespaces/using-codespaces-in-visual-studio.md
index fded97bd0166..5ee3ffdace4f 100644
--- a/translations/ru-RU/content/github/developing-online-with-codespaces/using-codespaces-in-visual-studio.md
+++ b/translations/ru-RU/content/github/developing-online-with-codespaces/using-codespaces-in-visual-studio.md
@@ -46,8 +46,12 @@ Connecting to {% data variables.product.prodname_github_codespaces %} with the
### Configuring a codespace for {% data variables.product.prodname_vs %}
-The default codespace environment created by {% data variables.product.prodname_vs %} includes popular frameworks and tools such as .NET Core, Microsoft SQL Server, Python, and the Windows SDK. {% data variables.product.prodname_github_codespaces %} created with {% data variables.product.prodname_vs %} can be customized through a subset of `devcontainers.json` properties and a new tool called devinit, included with {% data variables.product.prodname_vs %}.
+A codespace, created with {% data variables.product.prodname_vs %}, can be customized through a new tool called devinit, a command line tool included with {% data variables.product.prodname_vs %}.
#### devinit
-The [devinit](https://docs.microsoft.com/visualstudio/devinit/getting-started-with-devinit) command-line tool lets you install additional frameworks and tools into your Windows development codespaces, as well as run PowerShell scripts or modify environment variables. devinit supports a configuration file called [devinit.json](https://docs.microsoft.com/visualstudio/devinit/devinit-json), which can be added to your project for creating customized and repeatable development environments. For more information about Windows codespace configuration and devinit, see [Customize a codespace](https://docs.microsoft.com/visualstudio/ide/codespaces/customize-codespaces) in the {% data variables.product.prodname_vs %} documentation.
+[devinit](https://docs.microsoft.com/visualstudio/devinit/getting-started-with-devinit) lets you install additional frameworks and tools into your Windows development codespaces, modify environment variables, and more.
+
+devinit supports a configuration file called [devinit.json](https://docs.microsoft.com/visualstudio/devinit/devinit-json). You can add this file to your project if you want to create a customized and repeatable development environment. When you use devinit with a [devcontainer.json](https://docs.microsoft.com/visualstudio/ide/codespaces/customize-codespaces#running-devinit-when-creating-a-codespace) file, your codespaces will be automatically configured on creation.
+
+For more information about Windows codespace configuration and devinit, see [Customize a codespace](https://docs.microsoft.com/visualstudio/ide/codespaces/customize-codespaces) in the {% data variables.product.prodname_vs %} documentation. For more information about devinit, see [Getting started with devinit](https://docs.microsoft.com/visualstudio/devinit/getting-started-with-devinit).
diff --git a/translations/ru-RU/content/github/getting-started-with-github/create-a-repo.md b/translations/ru-RU/content/github/getting-started-with-github/create-a-repo.md
index 4d0dd3551a1d..20e8bd22c874 100644
--- a/translations/ru-RU/content/github/getting-started-with-github/create-a-repo.md
+++ b/translations/ru-RU/content/github/getting-started-with-github/create-a-repo.md
@@ -10,14 +10,26 @@ versions:
github-ae: '*'
---
+{% if currentVersion == "free-pro-team@latest" %}
+
You can store a variety of projects in {% data variables.product.product_name %} repositories, including open source projects. With [open source projects](http://opensource.org/about), you can share code to make better, more reliable software.
+{% elsif enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
+
+You can store a variety of projects in {% data variables.product.product_name %} repositories, including innersource projects. With innersource, you can share code to make better, more reliable software. For more information on innersource, see {% data variables.product.company_short %}'s whitepaper "[An introduction to innersource](https://resources.github.com/whitepapers/introduction-to-innersource/)."
+
+{% endif %}
+
+{% if currentVersion == "free-pro-team@latest" %}
+
{% note %}
**Note:** You can create public repositories for an open source project. When creating your public repository, make sure to include a [license file](http://choosealicense.com/) that determines how you want your project to be shared with others. {% data reusables.open-source.open-source-guide-repositories %} {% data reusables.open-source.open-source-learning-lab %}
{% endnote %}
+{% endif %}
+
{% data reusables.repositories.create_new %}
2. Type a short, memorable name for your repository. For example, "hello-world". 
3. Optionally, add a description of your repository. For example, "My first repository on
diff --git a/translations/ru-RU/content/github/getting-started-with-github/fork-a-repo.md b/translations/ru-RU/content/github/getting-started-with-github/fork-a-repo.md
index 11d83bcdbeb2..576060e664f2 100644
--- a/translations/ru-RU/content/github/getting-started-with-github/fork-a-repo.md
+++ b/translations/ru-RU/content/github/getting-started-with-github/fork-a-repo.md
@@ -25,10 +25,16 @@ For example, you can use forks to propose changes related to fixing a bug. Rathe
Open source software is based on the idea that by sharing code, we can make better, more reliable software. For more information, see the "[About the Open Source Initiative](http://opensource.org/about)" on the Open Source Initiative.
+For more information about applying open source principles to your organization's development work on {% data variables.product.product_location %}, see {% data variables.product.prodname_dotcom %}'s whitepaper "[An introduction to innersource](https://resources.github.com/whitepapers/introduction-to-innersource/)."
+
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
When creating your public repository from a fork of someone's project, make sure to include a license file that determines how you want your project to be shared with others. For more information, see "[Choose an open source license](http://choosealicense.com/)" at choosealicense.
{% data reusables.open-source.open-source-guide-repositories %} {% data reusables.open-source.open-source-learning-lab %}
+{% endif %}
+
{% note %}
**Note**: {% data reusables.repositories.desktop-fork %}
diff --git a/translations/ru-RU/content/github/getting-started-with-github/setting-up-a-trial-of-github-enterprise-server.md b/translations/ru-RU/content/github/getting-started-with-github/setting-up-a-trial-of-github-enterprise-server.md
index 77bf16419cdb..2f8261f76c36 100644
--- a/translations/ru-RU/content/github/getting-started-with-github/setting-up-a-trial-of-github-enterprise-server.md
+++ b/translations/ru-RU/content/github/getting-started-with-github/setting-up-a-trial-of-github-enterprise-server.md
@@ -36,7 +36,7 @@ To get the most out of your trial, follow these steps:
- [Quick start guide to {% data variables.product.prodname_dotcom %}](https://resources.github.com/webcasts/Quick-start-guide-to-GitHub/) webcast
- [Understanding the {% data variables.product.prodname_dotcom %} flow](https://guides.github.com/introduction/flow/) in {% data variables.product.prodname_dotcom %} Guides
- [Hello World](https://guides.github.com/activities/hello-world/) in {% data variables.product.prodname_dotcom %} Guides
-3. To configure your instance to meet your organization's needs, see "[Configuring your enterprise](/admin/configuration/configuring-your-enterprise)."
+3. To configure your instance to meet your organization's needs, see "[Configuring your enterprise](/enterprise/admin/configuration/configuring-your-enterprise)."
4. To integrate {% data variables.product.prodname_ghe_server %} with your identity provider, see "[Using SAML](/enterprise/admin/user-management/using-saml)" and "[Using LDAP](/enterprise/admin/authentication/using-ldap)."
5. Invite an unlimited number of people to join your trial.
- Add users to your {% data variables.product.prodname_ghe_server %} instance using built-in authentication or your configured identity provider. For more information, see "[Using built in authentication](/enterprise/admin/user-management/using-built-in-authentication)."
diff --git a/translations/ru-RU/content/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies.md b/translations/ru-RU/content/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies.md
index dacaed7bc19d..7e79109557bb 100644
--- a/translations/ru-RU/content/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies.md
+++ b/translations/ru-RU/content/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies.md
@@ -75,7 +75,7 @@ You can see all of the alerts that affect a particular project{% if currentVersi
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
By default, we notify people with admin permissions in the affected repositories about new
-{% data variables.product.prodname_dependabot_alerts %}.{% endif %} {% if currentVersion == "free-pro-team@latest" %}{% data variables.product.product_name %} never publicly discloses identified vulnerabilities for any repository. You can also make {% data variables.product.prodname_dependabot_alerts %} visible to additional people or teams working repositories that you own or have admin permissions for. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-dependabot-alerts)."
+{% data variables.product.prodname_dependabot_alerts %}.{% endif %} {% if currentVersion == "free-pro-team@latest" %}{% data variables.product.product_name %} never publicly discloses identified vulnerabilities for any repository. You can also make {% data variables.product.prodname_dependabot_alerts %} visible to additional people or teams working repositories that you own or have admin permissions for. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-security-alerts)."
{% endif %}
{% if enterpriseServerVersions contains currentVersion and currentVersion ver_lt "enterprise-server@2.22" %}
diff --git a/translations/ru-RU/content/github/managing-subscriptions-and-notifications-on-github/about-notifications.md b/translations/ru-RU/content/github/managing-subscriptions-and-notifications-on-github/about-notifications.md
index 337a63de5e0d..3c15a48c5431 100644
--- a/translations/ru-RU/content/github/managing-subscriptions-and-notifications-on-github/about-notifications.md
+++ b/translations/ru-RU/content/github/managing-subscriptions-and-notifications-on-github/about-notifications.md
@@ -21,7 +21,12 @@ You can choose to subscribe to notifications for:
- A conversation in a specific issue, pull request, or gist.
- All activity in a repository or team discussion.
- CI activity, such as the status of workflows in repositories set up with {% data variables.product.prodname_actions %}.
+{% if currentVersion == "free-pro-team@latest" %}
+- Issues, pulls requests, releases and discussions (if enabled) in a repository.
+{% endif %}
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}
- Releases in a repository.
+{% endif %}
You can also choose to automatically watch all repositories that you have push access to, except forks. You can watch any other repository you have access to manually by clicking **Watch**.
diff --git a/translations/ru-RU/content/github/managing-subscriptions-and-notifications-on-github/configuring-notifications.md b/translations/ru-RU/content/github/managing-subscriptions-and-notifications-on-github/configuring-notifications.md
index 0f18d8b62233..c2e7cb92cba8 100644
--- a/translations/ru-RU/content/github/managing-subscriptions-and-notifications-on-github/configuring-notifications.md
+++ b/translations/ru-RU/content/github/managing-subscriptions-and-notifications-on-github/configuring-notifications.md
@@ -21,16 +21,17 @@ versions:
### Notification delivery options
-You have three basic options for notification delivery:
- - the notifications inbox on {% data variables.product.product_name %}{% if currentVersion == "free-pro-team@latest" %}
- - the notifications inbox on {% data variables.product.prodname_mobile %}, which syncs with the inbox on {% data variables.product.product_name %}{% endif %}
- - an email client that uses a verified email address, which can also sync with the notifications inbox on {% data variables.product.product_name %}{% if currentVersion == "free-pro-team@latest" %} and {% data variables.product.prodname_mobile %}{% endif %}
+You can receive notifications for activity on {% data variables.product.product_name %} in the following locations.
+
+ - The notifications inbox in the {% data variables.product.product_name %} web interface{% if currentVersion == "free-pro-team@latest" %}
+ - The notifications inbox on {% data variables.product.prodname_mobile %}, which syncs with the inbox on {% data variables.product.product_name %}{% endif %}
+ - An email client that uses a verified email address, which can also sync with the notifications inbox on {% data variables.product.product_name %}{% if currentVersion == "free-pro-team@latest" %} and {% data variables.product.prodname_mobile %}{% endif %}
{% if currentVersion == "free-pro-team@latest" %}
{% data reusables.notifications-v2.notifications-inbox-required-setting %} For more information, see "[Choosing your notification settings](#choosing-your-notification-settings)."
{% endif %}
-{% data reusables.notifications-v2.tip-for-syncing-email-and-your-inbox-on-github %}
+{% data reusables.notifications.shared_state %}
#### Benefits of the notifications inbox
@@ -59,8 +60,21 @@ Email notifications also allow flexibility with the types of notifications you r
### About participating and watching notifications
-When you watch a repository, you're subscribing to updates for activity in that repository. Similarly, when you watch a specific team's discussions, you're subscribing to all conversation updates on that team's page. To see repositories that you're watching, see [https://github.com/watching](https://github.com/watching). For more information, see "[Managing subscriptions and notifications on GitHub](/github/managing-subscriptions-and-notifications-on-github/managing-subscriptions-for-activity-on-github)."
+When you watch a repository, you're subscribing to updates for activity in that repository. Similarly, when you watch a specific team's discussions, you're subscribing to all conversation updates on that team's page. For more information, see "[About team discussions](/github/building-a-strong-community/about-team-discussions)."
+
+To see repositories that you're watching, go to your [watching page](https://github.com/watching). For more information, see "[Managing subscriptions and notifications on GitHub](/github/managing-subscriptions-and-notifications-on-github/managing-subscriptions-for-activity-on-github)."
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}
+#### Configuring notifications
+{% endif %}
+You can configure notifications for a repository on the repository page, or on your watching page.
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %} You can choose to only receive notifications for releases in a repository, or ignore all notifications for a repository.{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+
+#### About custom notifications
+{% data reusables.notifications-v2.custom-notifications-beta %}
+You can customize notifications for a repository, for example, you can choose to only be notified when updates to one or more types of events (issues, pull request, releases, discussions) happen within a repository, or ignore all notifications for a repository.
+{% endif %} For more information, see "[Viewing your subscriptions](/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions#configuring-your-watch-settings-for-an-individual-repository)."
+#### Participating in conversations
Anytime you comment in a conversation or when someone @mentions your username, you are _participating_ in a conversation. By default, you are automatically subscribed to a conversation when you participate in it. You can unsubscribe from a conversation you've participated in manually by clicking **Unsubscribe** on the issue or pull request or through the **Unsubscribe** option in the notifications inbox.
For conversations you're watching or participating in, you can choose whether you want to receive notifications by email or through the notifications inbox on {% data variables.product.product_name %}{% if currentVersion == "free-pro-team@latest" %} and {% data variables.product.prodname_mobile %}{% endif %}.
@@ -95,7 +109,9 @@ Choose a default email address where you want to send updates for conversations
- Pull request pushes.
- Your own updates, such as when you open, comment on, or close an issue or pull request.
-Depending on the organization that owns the repository, you can also send notifications to different email addresses for specific repositories. For example, you can send notifications for a specific public repository to a verified personal email address. Your organization may require the email address to be verified for a specific domain. For more information, see “[Choosing where your organization’s email notifications are sent](/github/managing-subscriptions-and-notifications-on-github/configuring-notifications#choosing-where-your-organizations-email-notifications-are-sent)."
+Depending on the organization that owns the repository, you can also send notifications to different email addresses. Your organization may require the email address to be verified for a specific domain. For more information, see "[Choosing where your organization’s email notifications are sent](/github/managing-subscriptions-and-notifications-on-github/configuring-notifications#choosing-where-your-organizations-email-notifications-are-sent)."
+
+You can also send notifications for a specific repository to an email address. For more information, see "[About email notifications for pushes to your repository](/github/administering-a-repository/about-email-notifications-for-pushes-to-your-repository)."
{% data reusables.notifications-v2.email-notification-caveats %}
diff --git a/translations/ru-RU/content/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions.md b/translations/ru-RU/content/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions.md
index b038653bde09..4cb5408b6384 100644
--- a/translations/ru-RU/content/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions.md
+++ b/translations/ru-RU/content/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions.md
@@ -55,6 +55,13 @@ When you unwatch a repository, you unsubscribe from future updates from that rep
{% data reusables.notifications.access_notifications %}
1. In the left sidebar, under the list of repositories, use the "Manage notifications" drop-down to click **Watched repositories**. 
2. On the watched repositories page, after you've evaluated the repositories you're watching, choose whether to:
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}
- unwatch a repository
- only watch releases for a repository
- ignore all notifications for a repository
+{% endif %}
+{% if currentVersion == "free-pro-team@latest" %}
+ - unwatch a repository
+ - ignore all notifications for a repository
+ - customize the types of event you receive notifications for (issues, pull requests, releases or discussions, if enabled)
+{% endif %}
\ No newline at end of file
diff --git a/translations/ru-RU/content/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions.md b/translations/ru-RU/content/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions.md
index 80ead402f0e6..0c46df8cbbc6 100644
--- a/translations/ru-RU/content/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions.md
+++ b/translations/ru-RU/content/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions.md
@@ -32,7 +32,16 @@ When your inbox has too many notifications to manage, consider whether you have
For more information, see "[Configuring notifications](/github/managing-subscriptions-and-notifications-on-github/configuring-notifications#automatic-watching)."
-To see an overview of your repository subscriptions, see "[Reviewing repositories that you're watching](#reviewing-repositories-that-youre-watching)." Many people forget about repositories that they've chosen to watch in the past. From the "Watched repositories" page you can quickly unwatch repositories. For more information on ways to unsubscribe, see "[Managing subscriptions](/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions)."
+To see an overview of your repository subscriptions, see "[Reviewing repositories that you're watching](#reviewing-repositories-that-youre-watching)."
+{% if currentVersion == "free-pro-team@latest" %}
+{% tip %}
+
+**Tip:** You can select the types of event to be notified of by using the **Custom** option of the **Watch/Unwatch** dropdown list in your [watching page](https://github.com/watching) or on any repository page on {% data variables.product.prodname_dotcom_the_website %}. For more information, see "[Configuring your watch settings for an individual repository](#configuring-your-watch-settings-for-an-individual-repository)" below.
+
+{% endtip %}
+{% endif %}
+
+Many people forget about repositories that they've chosen to watch in the past. From the "Watched repositories" page you can quickly unwatch repositories. For more information on ways to unsubscribe, see "[Unwatch recommendations](https://github.blog/changelog/2020-11-10-unwatch-recommendations/)" on {% data variables.product.prodname_blog %} and "[Managing your subscriptions](/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions)." You can also create a triage worflow to help with the notifications you receive. For guidance on triage workflows, see "[Customizing a workflow for triaging your notifications](/github/managing-subscriptions-and-notifications-on-github/customizing-a-workflow-for-triaging-your-notifications)."
### Reviewing all of your subscriptions
@@ -55,20 +64,38 @@ To see an overview of your repository subscriptions, see "[Reviewing repositorie
### Reviewing repositories that you're watching
1. In the left sidebar, under the list of repositories, use the "Manage notifications" drop-down menu and click **Watched repositories**. 
-
-3. Evaluate the repositories that you are watching and decide if their updates are still relevant and helpful. When you watch a repository, you will be notified of all conversations for that repository.
-
+2. Evaluate the repositories that you are watching and decide if their updates are still relevant and helpful. When you watch a repository, you will be notified of all conversations for that repository.
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}

+{% endif %}
+{% if currentVersion == "free-pro-team@latest" %}
+ 
+{% endif %}
{% tip %}
- **Tip:** Instead of watching a repository, consider only watching releases for a repository or completely unwatching a repository. When you unwatch a repository, you can still be notified when you're @mentioned or participating in a thread. When you only watch releases for a repository, you're only notified when there's a new release, you're participating in a thread, or you or a team you're on is @mentioned.
+ **Tip:** Instead of watching a repository, consider only receiving notifications {% if currentVersion == "free-pro-team@latest" %}when there are updates to issues, pull requests, releases or discussions (if enabled for the repository), or any combination of these options,{% else %}for releases in a repository,{% endif %} or completely unwatching a repository.
+
+ When you unwatch a repository, you can still be notified when you're @mentioned or participating in a thread. When you configure to receive notifications for certain event types, you're only notified when there are updates to these event types in the repository, you're participating in a thread, or you or a team you're on is @mentioned.
{% endtip %}
### Configuring your watch settings for an individual repository
-You can choose whether to watch or unwatch an individual repository. You can also choose to only be notified of new releases or ignore an individual repository.
+You can choose whether to watch or unwatch an individual repository. You can also choose to only be notified of {% if currentVersion == "free-pro-team@latest" %}certain event types such as issues, pull requests, discussions (if enabled for the repository) and {% endif %}new releases, or completely ignore an individual repository.
{% data reusables.repositories.navigate-to-repo %}
-2. In the upper-right corner, click the "Watch" drop-down menu to select a watch option. 
+2. In the upper-right corner, click the "Watch" drop-down menu to select a watch option.
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}
+ 
+{% endif %}
+{% if currentVersion == "free-pro-team@latest" %}
+ 
+{% data reusables.notifications-v2.custom-notifications-beta %}
+The **Custom** option allows you to further customize notifications so that you're only notified when specific events happen in the repository, in addition to participating and @mentions.
+
+ 
+
+If you select "Issues", you will be notified about, and subscribed to, updates on every issue (including those that existed prior to you selecting this option) in the repository. If you're @mentioned in a pull request in this repository, you'll receive notifications for that too, and you'll be subscribed to updates on that specific pull request, in addition to being notified about issues.
+
+{% endif %}
diff --git a/translations/ru-RU/content/github/managing-your-work-on-github/creating-an-issue.md b/translations/ru-RU/content/github/managing-your-work-on-github/creating-an-issue.md
index d232f58950bb..71de245411d6 100644
--- a/translations/ru-RU/content/github/managing-your-work-on-github/creating-an-issue.md
+++ b/translations/ru-RU/content/github/managing-your-work-on-github/creating-an-issue.md
@@ -1,6 +1,7 @@
---
title: Creating an issue
intro: 'Issues can be used to keep track of bugs, enhancements, or other requests.'
+permissions: 'People with read permissions can create an issue in a repository where issues are enabled.'
redirect_from:
- /articles/creating-an-issue
versions:
@@ -9,8 +10,6 @@ versions:
github-ae: '*'
---
-{% data reusables.repositories.create-issue-in-public-repository %}
-
You can open a new issue based on code from an existing pull request. For more information, see "[Opening an issue from code](/github/managing-your-work-on-github/opening-an-issue-from-code)."
You can open a new issue directly from a comment in an issue or a pull request review. For more information, see "[Opening an issue from a comment](/github/managing-your-work-on-github/opening-an-issue-from-a-comment)."
diff --git a/translations/ru-RU/content/github/managing-your-work-on-github/opening-an-issue-from-a-comment.md b/translations/ru-RU/content/github/managing-your-work-on-github/opening-an-issue-from-a-comment.md
index c0417d832f18..0087d3f6aa2a 100644
--- a/translations/ru-RU/content/github/managing-your-work-on-github/opening-an-issue-from-a-comment.md
+++ b/translations/ru-RU/content/github/managing-your-work-on-github/opening-an-issue-from-a-comment.md
@@ -1,6 +1,7 @@
---
title: Opening an issue from a comment
intro: You can open a new issue from a specific comment in an issue or pull request.
+permissions: 'People with read permissions can create an issue in a repository where issues are enabled.'
versions:
free-pro-team: '*'
enterprise-server: '*'
@@ -9,6 +10,8 @@ versions:
When you open an issue from a comment, the issue contains a snippet showing where the comment was originally posted.
+{% data reusables.repositories.administrators-can-disable-issues %}
+
1. Navigate to the comment you'd like to open an issue from.
2. In that comment, click {% octicon "kebab-horizontal" aria-label="The horizontal kebab icon" %}. 
diff --git a/translations/ru-RU/content/github/managing-your-work-on-github/opening-an-issue-from-code.md b/translations/ru-RU/content/github/managing-your-work-on-github/opening-an-issue-from-code.md
index 4f75261f28fa..18519f57afac 100644
--- a/translations/ru-RU/content/github/managing-your-work-on-github/opening-an-issue-from-code.md
+++ b/translations/ru-RU/content/github/managing-your-work-on-github/opening-an-issue-from-code.md
@@ -1,6 +1,7 @@
---
title: Opening an issue from code
intro: You can open a new issue from a specific line or lines of code in a file or pull request.
+permissions: 'People with read permissions can create an issue in a repository where issues are enabled.'
redirect_from:
- /articles/opening-an-issue-from-code
versions:
@@ -13,7 +14,7 @@ When you open an issue from code, the issue contains a snippet showing the line

-{% data reusables.repositories.create-issue-in-public-repository %}
+{% data reusables.repositories.administrators-can-disable-issues %}
{% data reusables.repositories.navigate-to-repo %}
2. Locate the code you want to reference in an issue:
diff --git a/translations/ru-RU/content/github/managing-your-work-on-github/transferring-an-issue-to-another-repository.md b/translations/ru-RU/content/github/managing-your-work-on-github/transferring-an-issue-to-another-repository.md
index 87395c4b8357..7b0010fe5faa 100644
--- a/translations/ru-RU/content/github/managing-your-work-on-github/transferring-an-issue-to-another-repository.md
+++ b/translations/ru-RU/content/github/managing-your-work-on-github/transferring-an-issue-to-another-repository.md
@@ -11,7 +11,7 @@ versions:
To transfer an open issue to another repository, you must have write permissions on the repository the issue is in and the repository you're transferring the issue to. For more information, see "[Repository permission levels for an organization](/articles/repository-permission-levels-for-an-organization)."
-You can only transfer issues between repositories owned by the same user or organization account. You can't transfer an issue from a private repository to a public repository.
+You can only transfer issues between repositories owned by the same user or organization account.{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}You can't transfer an issue from a private repository to a public repository.{% endif %}
When you transfer an issue, comments and assignees are retained. The issue's labels and milestones are not retained. This issue will stay on any user-owned or organization-wide project boards and be removed from any repository project boards. For more information, see "[About project boards](/articles/about-project-boards)."
diff --git a/translations/ru-RU/content/github/searching-for-information-on-github/about-searching-on-github.md b/translations/ru-RU/content/github/searching-for-information-on-github/about-searching-on-github.md
index f48d3597b6da..ac24eb8d19c5 100644
--- a/translations/ru-RU/content/github/searching-for-information-on-github/about-searching-on-github.md
+++ b/translations/ru-RU/content/github/searching-for-information-on-github/about-searching-on-github.md
@@ -14,7 +14,7 @@ versions:
github-ae: '*'
---
-You can search globally across all of {% data variables.product.product_name %}, or scope your search to a particular repository or organization.
+{% data reusables.search.you-can-search-globally %}
- To search globally across all of {% data variables.product.product_name %}, type what you're looking for into the search field at the top of any page, and choose "All {% data variables.product.prodname_dotcom %}" in the search drop-down menu.
- To search within a particular repository or organization, navigate to the repository or organization page, type what you're looking for into the search field at the top of the page, and press **Enter**.
@@ -23,7 +23,8 @@ You can search globally across all of {% data variables.product.product_name %},
**Замечания:**
-- {% data reusables.search.required_login %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- {% data reusables.search.required_login %}{% endif %}
- {% data variables.product.prodname_pages %} sites are not searchable on {% data variables.product.product_name %}. However you can search the source content if it exists in the default branch of a repository, using code search. For more information, see "[Searching code](/articles/searching-code)." For more information about {% data variables.product.prodname_pages %}, see "[What is GitHub Pages?](/articles/what-is-github-pages/)"
- Currently our search doesn't support exact matching.
- Whenever you are searching in code files, only the first two results in each file will be returned.
@@ -36,7 +37,7 @@ After running a search on {% data variables.product.product_name %}, you can sor
### Types of searches on {% data variables.product.prodname_dotcom %}
-You can search the following types of information across all public {% data variables.product.product_name %} repositories, and all private {% data variables.product.product_name %} repositories you have access to:
+You can search for the following information across all repositories you can access on {% data variables.product.product_location %}.
- [Repositories](/articles/searching-for-repositories)
- [Topics](/articles/searching-topics)
diff --git a/translations/ru-RU/content/github/searching-for-information-on-github/searching-code.md b/translations/ru-RU/content/github/searching-for-information-on-github/searching-code.md
index c60f34f1ffc9..288cc76d9139 100644
--- a/translations/ru-RU/content/github/searching-for-information-on-github/searching-code.md
+++ b/translations/ru-RU/content/github/searching-for-information-on-github/searching-code.md
@@ -11,7 +11,7 @@ versions:
github-ae: '*'
---
-You can search for code globally across all of {% data variables.product.product_name %}, or search for code within a particular repository or organization. To search for code across all public repositories, you must be signed in to a {% data variables.product.product_name %} account. For more information, see "[About searching on GitHub](/articles/about-searching-on-github)."
+{% data reusables.search.you-can-search-globally %} For more information, see "[About searching on GitHub](/articles/about-searching-on-github)."
You can only search code using these code search qualifiers. Search qualifiers specifically for repositories, users, or commits, will not work when searching for code.
@@ -21,13 +21,13 @@ You can only search code using these code search qualifiers. Search qualifiers s
Due to the complexity of searching code, there are some restrictions on how searches are performed:
-- {% data reusables.search.required_login %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- {% data reusables.search.required_login %}{% endif %}
- Code in [forks](/articles/about-forks) is only searchable if the fork has more stars than the parent repository. Forks with fewer stars than the parent repository are **not** indexed for code search. To include forks with more stars than their parent in the search results, you will need to add `fork:true` or `fork:only` to your query. For more information, see "[Searching in forks](/articles/searching-in-forks)."
- Only the _default branch_ is indexed for code search.{% if currentVersion == "free-pro-team@latest" %}
- Only files smaller than 384 KB are searchable.{% else %}* Only files smaller than 5 MB are searchable.
- Only the first 500 KB of each file is searchable.{% endif %}
- Only repositories with fewer than 500,000 files are searchable.
-- Users who are signed in can search all public repositories.
- Except with [`filename`](#search-by-filename) searches, you must always include at least one search term when searching source code. For example, searching for [`language:javascript`](https://github.com/search?utf8=%E2%9C%93&q=language%3Ajavascript&type=Code&ref=searchresults) is not valid, while [`amazing language:javascript`](https://github.com/search?utf8=%E2%9C%93&q=amazing+language%3Ajavascript&type=Code&ref=searchresults) is.
- At most, search results can show two fragments from the same file, but there may be more results within the file.
- You can't use the following wildcard characters as part of your search query: . , : ; / \ ` ' " = * ! ? # $ & + ^ | ~ < > ( ) { } [ ]
. The search will simply ignore these symbols.
diff --git a/translations/ru-RU/content/github/searching-for-information-on-github/searching-commits.md b/translations/ru-RU/content/github/searching-for-information-on-github/searching-commits.md
index 7600b8fab038..8bbb3acb1622 100644
--- a/translations/ru-RU/content/github/searching-for-information-on-github/searching-commits.md
+++ b/translations/ru-RU/content/github/searching-for-information-on-github/searching-commits.md
@@ -96,14 +96,11 @@ To search commits in all repositories owned by a certain user or organization, u
| org:ORGNAME
| [**test org:github**](https://github.com/search?utf8=%E2%9C%93&q=test+org%3Agithub&type=Commits) matches commit messages with the word "test" in repositories owned by @github. |
| repo:USERNAME/REPO
| [**language repo:defunkt/gibberish**](https://github.com/search?utf8=%E2%9C%93&q=language+repo%3Adefunkt%2Fgibberish&type=Commits) matches commit messages with the word "language" in @defunkt's "gibberish" repository. |
-### Filter public or private repositories
+### Filter by repository visibility
-The `is` qualifier matches public or private commits.
+The `is` qualifier matches commits from repositories with the specified visibility. For more information, see "[About repository visibility](/github/creating-cloning-and-archiving-repositories/about-repository-visibility).
-| Qualifier | Пример |
-| ------------ | ------------------------------------------------------------------------------------------------ |
-| `is:public` | [**is:public**](https://github.com/search?q=is%3Apublic&type=Commits) matches public commits. |
-| `is:private` | [**is:private**](https://github.com/search?q=is%3Aprivate&type=Commits) matches private commits. |
+| Qualifier | Example | ------------- | ------------- |{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %} | `is:public` | [**is:public**](https://github.com/search?q=is%3Apublic&type=Commits) matches commits to public repositories.{% endif %} | `is:internal` | [**is:internal**](https://github.com/search?q=is%3Ainternal&type=Commits) matches commits to internal repositories. | `is:private` | [**is:private**](https://github.com/search?q=is%3Aprivate&type=Commits) matches commits to private repositories.
### Дополнительная литература
diff --git a/translations/ru-RU/content/github/searching-for-information-on-github/searching-for-repositories.md b/translations/ru-RU/content/github/searching-for-information-on-github/searching-for-repositories.md
index b7d384e6fdf9..e206422a64e9 100644
--- a/translations/ru-RU/content/github/searching-for-information-on-github/searching-for-repositories.md
+++ b/translations/ru-RU/content/github/searching-for-information-on-github/searching-for-repositories.md
@@ -10,7 +10,7 @@ versions:
github-ae: '*'
---
-You can search for repositories globally across all of {% data variables.product.product_name %}, or search for repositories within a particular organization. For more information, see "[About searching on {% data variables.product.prodname_dotcom %}](/articles/about-searching-on-github)."
+You can search for repositories globally across all of {% data variables.product.product_location %}, or search for repositories within a particular organization. For more information, see "[About searching on {% data variables.product.prodname_dotcom %}](/articles/about-searching-on-github)."
To include forks in the search results, you will need to add `fork:true` or `fork:only` to your query. For more information, see "[Searching in forks](/articles/searching-in-forks)."
@@ -20,22 +20,22 @@ To include forks in the search results, you will need to add `fork:true` or `for
With the `in` qualifier you can restrict your search to the repository name, repository description, contents of the README file, or any combination of these. When you omit this qualifier, only the repository name and description are searched.
-| Qualifier | Пример |
-| ----------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `in:name` | [**jquery in:name**](https://github.com/search?q=jquery+in%3Aname&type=Repositories) matches repositories with "jquery" in their name. |
-| `in:description` | [**jquery in:name,description**](https://github.com/search?q=jquery+in%3Aname%2Cdescription&type=Repositories) matches repositories with "jquery" in their name or description. |
-| `in:readme` | [**jquery in:readme**](https://github.com/search?q=jquery+in%3Areadme&type=Repositories) matches repositories mentioning "jquery" in their README file. |
-| `repo:owner/name` | [**repo:octocat/hello-world**](https://github.com/search?q=repo%3Aoctocat%2Fhello-world) matches a specific repository name. |
+| Qualifier | Пример |
+| ----------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `in:name` | [**jquery in:name**](https://github.com/search?q=jquery+in%3Aname&type=Repositories) matches repositories with "jquery" in the repository name. |
+| `in:description` | [**jquery in:name,description**](https://github.com/search?q=jquery+in%3Aname%2Cdescription&type=Repositories) matches repositories with "jquery" in the repository name or description. |
+| `in:readme` | [**jquery in:readme**](https://github.com/search?q=jquery+in%3Areadme&type=Repositories) matches repositories mentioning "jquery" in the repository's README file. |
+| `repo:owner/name` | [**repo:octocat/hello-world**](https://github.com/search?q=repo%3Aoctocat%2Fhello-world) matches a specific repository name. |
### Search based on the contents of a repository
-You can find a repository by searching for content in its README file, using the `in:readme` qualifier.
+You can find a repository by searching for content in the repository's README file using the `in:readme` qualifier. For more information, see "[About READMEs](/github/creating-cloning-and-archiving-repositories/about-readmes)."
Besides using `in:readme`, it's not possible to find repositories by searching for specific content within the repository. To search for a specific file or content within a repository, you can use the file finder or code-specific search qualifiers. For more information, see "[Finding files on {% data variables.product.prodname_dotcom %}](/articles/finding-files-on-github)" and "[Searching code](/articles/searching-code)."
-| Qualifier | Пример |
-| ----------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `in:readme` | [**octocat in:readme**](https://github.com/search?q=octocat+in%3Areadme&type=Repositories) matches repositories mentioning "octocat" in their README file. |
+| Qualifier | Пример |
+| ----------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `in:readme` | [**octocat in:readme**](https://github.com/search?q=octocat+in%3Areadme&type=Repositories) matches repositories mentioning "octocat" in the repository's README file. |
### Search within a user's or organization's repositories
@@ -48,7 +48,7 @@ To search in all repositories owned by a certain user or organization, you can u
### Search by repository size
-The `size` qualifier finds repositories that match a certain size (in kilobytes), using [greater than, less than, and range qualifiers](/articles/understanding-the-search-syntax).
+The `size` qualifier finds repositories that match a certain size (in kilobytes), using greater than, less than, and range qualifiers. For more information, see "[Understanding the search syntax](/github/searching-for-information-on-github/understanding-the-search-syntax)."
| Qualifier | Пример |
| ------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -59,7 +59,7 @@ The `size` qualifier finds repositories that match a certain size (in kilobytes)
### Search by number of followers
-You can filter repositories based on the number of followers that they have, using the `followers` qualifier with [greater than, less than, and range qualifiers](/articles/understanding-the-search-syntax).
+You can filter repositories based on the number of users who follow the repositories, using the `followers` qualifier with greater than, less than, and range qualifiers. For more information, see "[Understanding the search syntax](/github/searching-for-information-on-github/understanding-the-search-syntax)."
| Qualifier | Пример |
| ------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -68,7 +68,7 @@ You can filter repositories based on the number of followers that they have, usi
### Search by number of forks
-The `forks` qualifier specifies the number of forks a repository should have, using [greater than, less than, and range qualifiers](/articles/understanding-the-search-syntax).
+The `forks` qualifier specifies the number of forks a repository should have, using greater than, less than, and range qualifiers. For more information, see "[Understanding the search syntax](/github/searching-for-information-on-github/understanding-the-search-syntax)."
| Qualifier | Пример |
| ------------------------- | ----------------------------------------------------------------------------------------------------------------------------------------- |
@@ -79,7 +79,7 @@ The `forks` qualifier specifies the number of forks a repository should have, us
### Search by number of stars
-You can search repositories based on the number of [stars](/articles/saving-repositories-with-stars) a repository has, using [greater than, less than, and range qualifiers](/articles/understanding-the-search-syntax)
+You can search repositories based on the number of stars the repositories have, using greater than, less than, and range qualifiers. For more information, see "[Saving repositories with stars](/github/getting-started-with-github/saving-repositories-with-stars)" and "[Understanding the search syntax](/github/searching-for-information-on-github/understanding-the-search-syntax)."
| Qualifier | Пример |
| ------------------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -103,7 +103,7 @@ Both take a date as a parameter. {% data reusables.time_date.date_format %} {% d
### Search by language
-You can search repositories based on the main language they're written in.
+You can search repositories based on the language of the code in the repositories.
| Qualifier | Пример |
| ------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -111,7 +111,7 @@ You can search repositories based on the main language they're written in.
### Search by topic
-You can find all of the repositories that are classified with a particular [topic](/articles/classifying-your-repository-with-topics).
+You can find all of the repositories that are classified with a particular topic. For more information, see "[Classifying your repository with topics](/github/administering-a-repository/classifying-your-repository-with-topics)."
| Qualifier | Пример |
| ------------------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -119,35 +119,36 @@ You can find all of the repositories that are classified with a particular [topi
### Search by number of topics
-You can search repositories by the number of [topics](/articles/classifying-your-repository-with-topics) that have been applied to them, using the `topics` qualifier along with [greater than, less than, and range qualifiers](/articles/understanding-the-search-syntax).
+You can search repositories by the number of topics that have been applied to the repositories, using the `topics` qualifier along with greater than, less than, and range qualifiers. For more information, see "[Classifying your repository with topics](/github/administering-a-repository/classifying-your-repository-with-topics)" and "[Understanding the search syntax](/github/searching-for-information-on-github/understanding-the-search-syntax)."
| Qualifier | Пример |
| -------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| topics:n
| [**topics:5**](https://github.com/search?utf8=%E2%9C%93&q=topics%3A5&type=Repositories&ref=searchresults) matches repositories that have five topics. |
| | [**topics:>3**](https://github.com/search?utf8=%E2%9C%93&q=topics%3A%3E3&type=Repositories&ref=searchresults) matches repositories that have more than three topics. |
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
### Search by license
-You can search repositories by their [license](/articles/licensing-a-repository). You must use a [license keyword](/articles/licensing-a-repository/#searching-github-by-license-type) to filter repositories by a particular license or license family.
+You can search repositories by the type of license in the repositories. You must use a license keyword to filter repositories by a particular license or license family. For more information, see "[Licensing a repository](/github/creating-cloning-and-archiving-repositories/licensing-a-repository)."
| Qualifier | Пример |
| -------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| license:LICENSE_KEYWORD
| [**license:apache-2.0**](https://github.com/search?utf8=%E2%9C%93&q=license%3Aapache-2.0&type=Repositories&ref=searchresults) matches repositories that are licensed under Apache License 2.0. |
-### Search by public or private repository
+{% endif %}
+
+### Search by repository visibility
-You can filter your search based on whether a repository is public or private.
+You can filter your search based on the visibility of the repositories. For more information, see "[About repository visibility](/github/creating-cloning-and-archiving-repositories/about-repository-visibility)."
-| Qualifier | Пример |
-| ------------ | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
-| `is:public` | [**is:public org:github**](https://github.com/search?q=is%3Apublic+org%3Agithub&type=Repositories&utf8=%E2%9C%93) matches repositories owned by GitHub that are public. |
-| `is:private` | [**is:private pages**](https://github.com/search?utf8=%E2%9C%93&q=pages+is%3Aprivate&type=Repositories) matches private repositories you have access to and that contain the word "pages." |
+| Qualifier | Example | ------------- | ------------- |{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %} | `is:public` | [**is:public org:github**](https://github.com/search?q=is%3Apublic+org%3Agithub&type=Repositories) matches public repositories owned by {% data variables.product.company_short %}.{% endif %} | `is:internal` | [**is:internal test**](https://github.com/search?q=is%3Ainternal+test&type=Repositories) matches internal repositories that you can access and contain the word "test". | `is:private` | [**is:private pages**](https://github.com/search?q=is%3Aprivate+pages&type=Repositories) matches private repositories that you can access and contain the word "pages."
{% if currentVersion == "free-pro-team@latest" %}
### Search based on whether a repository is a mirror
-You can search repositories based on whether or not they're a mirror and are hosted elsewhere. For more information, see "[Finding ways to contribute to open source on {% data variables.product.prodname_dotcom %}](/github/getting-started-with-github/finding-ways-to-contribute-to-open-source-on-github)."
+You can search repositories based on whether the repositories are mirrors and hosted elsewhere. For more information, see "[Finding ways to contribute to open source on {% data variables.product.prodname_dotcom %}](/github/getting-started-with-github/finding-ways-to-contribute-to-open-source-on-github)."
| Qualifier | Пример |
| -------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -158,7 +159,7 @@ You can search repositories based on whether or not they're a mirror and are hos
### Search based on whether a repository is archived
-You can search repositories based on whether or not they're [archived](/articles/about-archiving-repositories).
+You can search repositories based on whether or not the repositories are archived. For more information, see "[About archiving repositories](/github/creating-cloning-and-archiving-repositories/about-archiving-repositories)."
| Qualifier | Пример |
| ---------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -166,6 +167,7 @@ You can search repositories based on whether or not they're [archived](/articles
| `archived:false` | [**archived:false GNOME**](https://github.com/search?utf8=%E2%9C%93&q=archived%3Afalse+GNOME&type=) matches repositories that are not archived and contain the word "GNOME." |
{% if currentVersion == "free-pro-team@latest" %}
+
### Search based on number of issues with `good first issue` or `help wanted` labels
You can search for repositories that have a minimum number of issues labeled `help-wanted` or `good-first-issue` with the qualifiers `help-wanted-issues:>n` and `good-first-issues:>n`. For more information, see "[Encouraging helpful contributions to your project with labels](/github/building-a-strong-community/encouraging-helpful-contributions-to-your-project-with-labels)."
@@ -174,6 +176,7 @@ You can search for repositories that have a minimum number of issues labeled `he
| -------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
| `good-first-issues:>n` | [**good-first-issues:>2 javascript**](https://github.com/search?utf8=%E2%9C%93&q=javascript+good-first-issues%3A%3E2&type=) matches repositories with more than two issues labeled `good-first-issue` and that contain the word "javascript." |
| `help-wanted-issues:>n` | [**help-wanted-issues:>4 react**](https://github.com/search?utf8=%E2%9C%93&q=react+help-wanted-issues%3A%3E4&type=) matches repositories with more than four issues labeled `help-wanted` and that contain the word "React." |
+
{% endif %}
### Дополнительная литература
diff --git a/translations/ru-RU/content/github/searching-for-information-on-github/searching-issues-and-pull-requests.md b/translations/ru-RU/content/github/searching-for-information-on-github/searching-issues-and-pull-requests.md
index 0febc54844e7..8a62bcf03395 100644
--- a/translations/ru-RU/content/github/searching-for-information-on-github/searching-issues-and-pull-requests.md
+++ b/translations/ru-RU/content/github/searching-for-information-on-github/searching-issues-and-pull-requests.md
@@ -64,14 +64,11 @@ You can filter issues and pull requests based on whether they're open or closed
| `is:open` | [**performance is:open is:issue**](https://github.com/search?q=performance+is%3Aopen+is%3Aissue&type=Issues) matches open issues with the word "performance." |
| `is:closed` | [**android is:closed**](https://github.com/search?utf8=%E2%9C%93&q=android+is%3Aclosed&type=) matches closed issues and pull requests with the word "android." |
-### Search by public or private repository
+### Filter by repository visibility
-If you're [searching across all of {% data variables.product.product_name %}](https://github.com/search), it can be helpful to filter your results based on whether the repository is public or private. You can do this with `is:public` and `is:private`.
+You can filter by the visibility of the repository containing the issues and pull requests using the `is` qualifier. For more information, see "[About repository visibility](/github/creating-cloning-and-archiving-repositories/about-repository-visibility)."
-| Qualifier | Пример |
-| ------------ | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `is:public` | [**is:public**](https://github.com/search?q=is%3Apublic&type=Issues) matches issues and pull requests in all public repositories. |
-| `is:private` | [**is:private cupcake**](https://github.com/search?q=is%3Aprivate&type=Issues) matches issues and pull requests that contain the word "cupcake" in private repositories you have access to. |
+| Qualifier | Example | ------------- | ------------- |{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %} | `is:public` | [**is:public**](https://github.com/search?q=is%3Apublic&type=Issues) matches issues and pull requests in public repositories.{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.20" or currentVersion == "github-ae@latest" %} | `is:internal` | [**is:internal**](https://github.com/search?q=is%3Ainternal&type=Issues) matches issues and pull requests in internal repositories.{% endif %} | `is:private` | [**is:private cupcake**](https://github.com/search?q=is%3Aprivate+cupcake&type=Issues) matches issues and pull requests that contain the word "cupcake" in private repositories you can access.
### Search by author
diff --git a/translations/ru-RU/content/github/setting-up-and-managing-organizations-and-teams/about-your-organization-dashboard.md b/translations/ru-RU/content/github/setting-up-and-managing-organizations-and-teams/about-your-organization-dashboard.md
index 4182fd11b568..fe9be4889c25 100644
--- a/translations/ru-RU/content/github/setting-up-and-managing-organizations-and-teams/about-your-organization-dashboard.md
+++ b/translations/ru-RU/content/github/setting-up-and-managing-organizations-and-teams/about-your-organization-dashboard.md
@@ -37,8 +37,8 @@ For instance, the organization news feed shows updates when someone in the organ
- Submits a pull request review comment.
- Forks a repository.
- Creates a wiki page.
- - Pushes commits.
- - Creates a public repository.
+ - Pushes commits.{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+ - Creates a public repository.{% endif %}
### Further information
diff --git a/translations/ru-RU/content/github/setting-up-and-managing-organizations-and-teams/restricting-repository-visibility-changes-in-your-organization.md b/translations/ru-RU/content/github/setting-up-and-managing-organizations-and-teams/restricting-repository-visibility-changes-in-your-organization.md
index bbb35db9fdf4..fcc618c5037e 100644
--- a/translations/ru-RU/content/github/setting-up-and-managing-organizations-and-teams/restricting-repository-visibility-changes-in-your-organization.md
+++ b/translations/ru-RU/content/github/setting-up-and-managing-organizations-and-teams/restricting-repository-visibility-changes-in-your-organization.md
@@ -9,7 +9,7 @@ versions:
github-ae: '*'
---
-You can restrict the ability to change repository visibility to organization owners only, or allow members with admin privileges for a repository to also change visibility from private to public or public to private.
+You can restrict the ability to change repository visibility to organization owners only, or allow members with admin privileges for a repository to also change visibility.
{% warning %}
@@ -24,3 +24,7 @@ You can restrict the ability to change repository visibility to organization own
{% data reusables.organizations.member-privileges %}
5. Under "Repository visibility change", deselect **Allow members to change repository visibilities for this organization**. 
6. Click **Save**.
+
+### Дополнительная литература
+
+- "[About repository visibility](/github/creating-cloning-and-archiving-repositories/about-repository-visibility)"
diff --git a/translations/ru-RU/content/github/setting-up-and-managing-organizations-and-teams/reviewing-the-audit-log-for-your-organization.md b/translations/ru-RU/content/github/setting-up-and-managing-organizations-and-teams/reviewing-the-audit-log-for-your-organization.md
index 40bf0acf30a0..45b8372a5b6a 100644
--- a/translations/ru-RU/content/github/setting-up-and-managing-organizations-and-teams/reviewing-the-audit-log-for-your-organization.md
+++ b/translations/ru-RU/content/github/setting-up-and-managing-organizations-and-teams/reviewing-the-audit-log-for-your-organization.md
@@ -1,6 +1,7 @@
---
title: Reviewing the audit log for your organization
intro: 'The audit log allows organization admins to quickly review the actions performed by members of your organization. It includes details such as who performed the action, what the action was, and when it was performed.'
+miniTocMaxHeadingLevel: 4
redirect_from:
- /articles/reviewing-the-audit-log-for-your-organization
versions:
@@ -11,7 +12,7 @@ versions:
### Accessing the audit log
-The audit log lists actions performed within the last 90 days. Only owners can access an organization's audit log.
+The audit log lists events triggered by activities that affect your organization within the last 90 days. Only owners can access an organization's audit log.
{% data reusables.profile.access_profile %}
{% data reusables.profile.access_org %}
@@ -26,73 +27,111 @@ The audit log lists actions performed within the last 90 days. Only owners can a
To search for specific events, use the `action` qualifier in your query. Actions listed in the audit log are grouped within the following categories:
-| Category Name | Description |
-| ------------------------------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |{% if currentVersion == "free-pro-team@latest" %}
-| `учетная запись` | Contains all activities related to your organization account.{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-| `выставление счетов` | Contains all activities related to your organization's billing.{% endif %}
-| `discussion_post` | Contains all activities related to discussions posted to a team page. |
-| `discussion_post_reply` | Contains all activities related to replies to discussions posted to a team page. |
-| `перехватчик` | Contains all activities related to webhooks. |
-| `integration_installation_request` | Contains all activities related to organization member requests for owners to approve integrations for use in the organization. |{% if currentVersion == "free-pro-team@latest" %}
-| `marketplace_agreement_signature` | Contains all activities related to signing the {% data variables.product.prodname_marketplace %} Developer Agreement. |
-| `marketplace_listing` | Contains all activities related to listing apps in {% data variables.product.prodname_marketplace %}.{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
-| `members_can_create_pages` | Contains all activities related to disabling the publication of {% data variables.product.prodname_pages %} sites for repositories in the organization. For more information, see "[Restricting publication of {% data variables.product.prodname_pages %} sites for your organization](/github/setting-up-and-managing-organizations-and-teams/disabling-publication-of-github-pages-sites-for-your-organization)." |{% endif %}
-| `org` | Contains all activities related to organization membership{% if currentVersion == "free-pro-team@latest" %}
-| `org_credential_authorization` | Contains all activities related to authorizing credentials for use with SAML single sign-on.{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "github-ae@latest" %}
-| `organization_label` | Contains all activities related to default labels for repositories in your organization.{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-| `payment_method` | Contains all activities related to how your organization pays for GitHub.{% endif %}
-| `profile_picture` | Contains all activities related to your organization's profile picture. |
-| `проект` | Contains all activities related to project boards. |
-| `protected_branch` | Contains all activities related to protected branches. |
-| `repo` | Contains all activities related to the repositories owned by your organization.{% if currentVersion == "free-pro-team@latest" %}
-| `repository_content_analysis` | Contains all activities related to [enabling or disabling data use for a private repository](/articles/about-github-s-use-of-your-data). |
-| `repository_dependency_graph` | Contains all activities related to [enabling or disabling the dependency graph for a private repository](/github/visualizing-repository-data-with-graphs/exploring-the-dependencies-and-dependents-of-a-repository).{% endif %}{% if currentVersion != "github-ae@latest" %}
-| `repository_vulnerability_alert` | Contains all activities related to [{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot %}{% else %}security{% endif %} alerts for vulnerable dependencies](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies).{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-| `sponsors` | Contains all events related to sponsor buttons (see "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)"){% endif %}{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-| `команда` | Contains all activities related to teams in your organization.{% endif %}
-| `team_discussions` | Contains activities related to managing team discussions for an organization. |
+| Category name | Description |
+| ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |{% if currentVersion == "free-pro-team@latest" %}
+| [`учетная запись`](#account-category-actions) | Contains all activities related to your organization account. |
+| [`advisory_credit`](#advisory_credit-category-actions) | Contains all activities related to crediting a contributor for a security advisory in the {% data variables.product.prodname_advisory_database %}. For more information, see "[About {% data variables.product.prodname_dotcom %} Security Advisories](/github/managing-security-vulnerabilities/about-github-security-advisories)." |
+| [`выставление счетов`](#billing-category-actions) | Contains all activities related to your organization's billing. |
+| [`dependabot_alerts`](#dependabot_alerts-category-actions) | Contains organization-level configuration activities for {% data variables.product.prodname_dependabot %} alerts in existing repositories. For more information, see "[About alerts for vulnerable dependencies](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies)." |
+| [`dependabot_alerts_new_repos`](#dependabot_alerts_new_repos-category-actions) | Contains organization-level configuration activities for {% data variables.product.prodname_dependabot %} alerts in new repositories created in the organization. |
+| [`dependabot_security_updates`](#dependabot_security_updates-category-actions) | Contains organization-level configuration activities for {% data variables.product.prodname_dependabot_security_updates %} in existing repositories. For more information, see "[Configuring {% data variables.product.prodname_dependabot_security_updates %}](/github/managing-security-vulnerabilities/configuring-dependabot-security-updates)." |
+| [`dependabot_security_updates_new_repos`](#dependabot_security_updates_new_repos-category-actions) | Contains organization-level configuration activities for {% data variables.product.prodname_dependabot_security_updates %} for new repositories created in the organization. |
+| [`dependency_graph`](#dependency_graph-category-actions) | Contains organization-level configuration activities for dependency graphs for repositories. For more information, see "[About the dependency graph](/github/visualizing-repository-data-with-graphs/about-the-dependency-graph)." |
+| [`dependency_graph_new_repos`](#dependency_graph_new_repos-category-actions) | Contains organization-level configuration activities for new repositories created in the organization.{% endif %}
+| [`discussion_post`](#discussion_post-category-actions) | Contains all activities related to discussions posted to a team page. |
+| [`discussion_post_reply`](#discussion_post_reply-category-actions) | Contains all activities related to replies to discussions posted to a team page. |
+| [`перехватчик`](#hook-category-actions) | Contains all activities related to webhooks. |
+| [`integration_installation_request`](#integration_installation_request-category-actions) | Contains all activities related to organization member requests for owners to approve integrations for use in the organization. |
+| [`проблема`](#issue-category-actions) | Contains activities related to deleting an issue. |{% if currentVersion == "free-pro-team@latest" %}
+| [`marketplace_agreement_signature`](#marketplace_agreement_signature-category-actions) | Contains all activities related to signing the {% data variables.product.prodname_marketplace %} Developer Agreement. |
+| [`marketplace_listing`](#marketplace_listing-category-actions) | Contains all activities related to listing apps in {% data variables.product.prodname_marketplace %}.{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
+| [`members_can_create_pages`](#members_can_create_pages-category-actions) | Contains all activities related to disabling the publication of {% data variables.product.prodname_pages %} sites for repositories in the organization. For more information, see "[Restricting publication of {% data variables.product.prodname_pages %} sites for your organization](/github/setting-up-and-managing-organizations-and-teams/disabling-publication-of-github-pages-sites-for-your-organization)." |{% endif %}
+| [`org`](#org-category-actions) | Contains activities related to organization membership.{% if currentVersion == "free-pro-team@latest" %}
+| [`org_credential_authorization`](#org_credential_authorization-category-actions) | Contains all activities related to authorizing credentials for use with SAML single sign-on.{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "github-ae@latest" %}
+| [`organization_label`](#organization_label-category-actions) | Contains all activities related to default labels for repositories in your organization.{% endif %}
+| [`oauth_application`](#oauth_application-category-actions) | Contains all activities related to OAuth Apps. |{% if currentVersion == "free-pro-team@latest" %}
+| [`payment_method`](#payment_method-category-actions) | Contains all activities related to how your organization pays for GitHub.{% endif %}
+| [`profile_picture`](#profile_picture-category-actions) | Contains all activities related to your organization's profile picture. |
+| [`проект`](#project-category-actions) | Contains all activities related to project boards. |
+| [`protected_branch`](#protected_branch-category-actions) | Contains all activities related to protected branches. |
+| [`repo`](#repo-category-actions) | Contains activities related to the repositories owned by your organization.{% if currentVersion == "free-pro-team@latest" %}
+| [`repository_advisory`](#repository_advisory-category-actions) | Contains repository-level activities related to security advisories in the {% data variables.product.prodname_advisory_database %}. For more information, see "[About {% data variables.product.prodname_dotcom %} Security Advisories](/github/managing-security-vulnerabilities/about-github-security-advisories)." |
+| [`repository_content_analysis`](#repository_content_analysis-category-actions) | Contains all activities related to [enabling or disabling data use for a private repository](/articles/about-github-s-use-of-your-data).{% endif %}{% if currentVersion != "github-ae@latest" %}
+| [`repository_dependency_graph`](#repository_dependency_graph-category-actions) | Contains repository-level activities related to enabling or disabling the dependency graph for a |
+| {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repository. For more information, see "[About the dependency graph](/github/visualizing-repository-data-with-graphs/about-the-dependency-graph)."{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %} | |
+| [`repository_secret_scanning`](#repository_secret_scanning-category-actions) | Contains repository-level activities related to secret scanning. For more information, see "[About secret scanning](/github/administering-a-repository/about-secret-scanning)." |{% endif %}{% if currentVersion != "github-ae@latest" %}
+| [`repository_vulnerability_alert`](#repository_vulnerability_alert-category-actions) | Contains all activities related to [{% data variables.product.prodname_dependabot_alerts %} for vulnerable dependencies](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies).{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| [`repository_vulnerability_alerts`](#repository_vulnerability_alerts-category-actions) | Contains repository-level configuration activities for {% data variables.product.prodname_dependabot %} alerts. |{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %}
+| [`secret_scanning`](#secret_scanning-category-actions) | Contains organization-level configuration activities for secret scanning in existing repositories. For more information, see "[About secret scanning](/github/administering-a-repository/about-secret-scanning)." |
+| [`secret_scanning_new_repos`](#secret_scanning_new_repos-category-actions) | Contains organization-level configuration activities for secret scanning for new repositories created in the organization. |{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| [`sponsors`](#sponsors-category-actions) | Contains all events related to sponsor buttons (see "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)"){% endif %}{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
+| [`команда`](#team-category-actions) | Contains all activities related to teams in your organization.{% endif %}
+| [`team_discussions`](#team_discussions-category-actions) | Contains activities related to managing team discussions for an organization. |
You can search for specific sets of actions using these terms. Например:
* `action:team` finds all events grouped within the team category.
* `-action:hook` excludes all events in the webhook category.
-Each category has a set of associated events that you can filter on. Например:
+Each category has a set of associated actions that you can filter on. Например:
* `action:team.create` finds all events where a team was created.
* `-action:hook.events_changed` excludes all events where the events on a webhook have been altered.
-This list describes the available categories and associated events:
-
-{% if currentVersion == "free-pro-team@latest" %}- [The `account` category](#the-account-category)
-- [The `billing` category](#the-billing-category){% endif %}
-- [The `discussion_post` category](#the-discussion_post-category)
-- [The `discussion_post_reply` category](#the-discussion_post_reply-category)
-- [The `hook` category](#the-hook-category)
-- [The `integration_installation_request` category](#the-integration_installation_request-category)
-- [The `issue` category](#the-issue-category){% if currentVersion == "free-pro-team@latest" %}
-- [The `marketplace_agreement_signature` category](#the-marketplace_agreement_signature-category)
-- [The `marketplace_listing` category](#the-marketplace_listing-category){% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
-- [The `members_can_create_pages` category](#the-members_can_create_pages-category){% endif %}
-- [The `org` category](#the-org-category){% if currentVersion == "free-pro-team@latest" %}
-- [The `org_credential_authorization` category](#the-org_credential_authorization-category){% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "github-ae@latest" %}
-- [The `organization_label` category](#the-organization_label-category){% endif %}
-- [The `oauth_application` category](#the-oauth_application-category){% if currentVersion == "free-pro-team@latest" %}
-- [The `payment_method` category](#the-payment_method-category){% endif %}
-- [The `profile_picture` category](#the-profile_picture-category)
-- [The `project` category](#the-project-category)
-- [The `protected_branch` category](#the-protected_branch-category)
-- [The `repo` category](#the-repo-category){% if currentVersion == "free-pro-team@latest" %}
-- [The `repository_content_analysis` category](#the-repository_content_analysis-category)
-- [The `repository_dependency_graph` category](#the-repository_dependency_graph-category){% endif %}{% if currentVersion != "github-ae@latest" %}
-- [The `repository_vulnerability_alert` category](#the-repository_vulnerability_alert-category){% endif %}{% if currentVersion == "free-pro-team@latest" %}
-- [The `sponsors` category](#the-sponsors-category){% endif %}{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-- [The `team` category](#the-team-category){% endif %}
-- [The `team_discussions` category](#the-team_discussions-category)
+#### Search based on time of action
+
+Use the `created` qualifier to filter events in the audit log based on when they occurred. {% data reusables.time_date.date_format %} {% data reusables.time_date.time_format %}
+
+{% data reusables.search.date_gt_lt %} For example:
+
+ * `created:2014-07-08` finds all events that occurred on July 8th, 2014.
+ * `created:>=2014-07-08` finds all events that occurred on or after July 8th, 2014.
+ * `created:<=2014-07-08` finds all events that occurred on or before July 8th, 2014.
+ * `created:2014-07-01..2014-07-31` finds all events that occurred in the month of July 2014.
+
+The audit log contains data for the past 90 days, but you can use the `created` qualifier to search for events earlier than that.
+
+#### Search based on location
+
+Using the qualifier `country`, you can filter events in the audit log based on the originating country. You can use a country's two-letter short code or its full name. Keep in mind that countries with spaces in their name will need to be wrapped in quotation marks. Например:
+
+ * `country:de` finds all events that occurred in Germany.
+ * `country:Mexico` finds all events that occurred in Mexico.
+ * `country:"United States"` all finds events that occurred in the United States.
+
+{% if currentVersion == "free-pro-team@latest" %}
+### Exporting the audit log
+
+{% data reusables.audit_log.export-log %}
+{% data reusables.audit_log.exported-log-keys-and-values %}
+{% endif %}
+
+### Using the Audit log API
+
+{% note %}
+
+**Note**: The Audit log API is available for organizations using {% data variables.product.prodname_enterprise %}. {% data reusables.gated-features.more-info-org-products %}
+
+{% endnote %}
+
+To ensure a secure IP and maintain compliance for your organization, you can use the Audit log API to keep copies of your audit log data and monitor:
+* Access to your organization or repository settings.
+* Changes in permissions.
+* Added or removed users in an organization, repository, or team.
+* Users being promoted to admin.
+* Changes to permissions of a GitHub App.
+
+The GraphQL response can include data for up to 90 to 120 days.
+
+For example, you can make a GraphQL request to see all the new organization members added to your organization. For more information, see the "[GraphQL API Audit Log](/v4/interface/auditentry/)."
+
+### Audit log actions
+
+An overview of some of the most common actions that are recorded as events in the audit log.
{% if currentVersion == "free-pro-team@latest" %}
-##### The `account` category
+#### `account` category actions
| Действие | Description |
| ----------------------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -101,30 +140,81 @@ This list describes the available categories and associated events:
| `pending_plan_change` | Triggered when an organization owner or billing manager [cancels or downgrades a paid subscription](/articles/how-does-upgrading-or-downgrading-affect-the-billing-process/). |
| `pending_subscription_change` | Triggered when a [{% data variables.product.prodname_marketplace %} free trial starts or expires](/articles/about-billing-for-github-marketplace/). |
-##### The `billing` category
+#### `advisory_credit` category actions
+
+| Действие | Description |
+| --------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| `accept` | Triggered when someone accepts credit for a security advisory. For more information, see "[Editing a security advisory](/github/managing-security-vulnerabilities/editing-a-security-advisory)." |
+| `create` | Triggered when the administrator of a security advisory adds someone to the credit section. |
+| `decline` | Triggered when someone declines credit for a security advisory. |
+| `destroy` | Triggered when the administrator of a security advisory removes someone from the credit section. |
+
+#### `billing` category actions
| Действие | Description |
| --------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------ |
| `change_billing_type` | Triggered when your organization [changes how it pays for {% data variables.product.prodname_dotcom %}](/articles/adding-or-editing-a-payment-method). |
| `change_email` | Triggered when your organization's [billing email address](/articles/setting-your-billing-email) changes. |
+#### `dependabot_alerts` category actions
+
+| Действие | Description |
+| ----------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| `отключить` | Triggered when an organization owner disables {% data variables.product.prodname_dependabot_alerts %} for all existing {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repositories. For more information, see "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)." |
+| `включить` | Triggered when an organization owner enables {% data variables.product.prodname_dependabot_alerts %} for all existing {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repositories. |
+
+#### `dependabot_alerts_new_repos` category actions
+
+| Действие | Description |
+| ----------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `отключить` | Triggered when an organization owner disables {% data variables.product.prodname_dependabot_alerts %} for all new {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repositories. For more information, see "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)." |
+| `включить` | Triggered when an organization owner enbles {% data variables.product.prodname_dependabot_alerts %} for all new {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repositories. |
+
+#### `dependabot_security_updates` category actions
+
+| Действие | Description |
+| ----------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `отключить` | Triggered when an organization owner disables {% data variables.product.prodname_dependabot_security_updates %} for all existing repositories. For more information, see "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)." |
+| `включить` | Triggered when an organization owner enables {% data variables.product.prodname_dependabot_security_updates %} for all existing repositories. |
+
+#### `dependabot_security_updates_new_repos` category actions
+
+| Действие | Description |
+| ----------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `отключить` | Triggered when an organization owner disables {% data variables.product.prodname_dependabot_security_updates %} for all new repositories. For more information, see "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)." |
+| `включить` | Triggered when an organization owner enables {% data variables.product.prodname_dependabot_security_updates %} for all new repositories. |
+
+#### `dependency_graph` category actions
+
+| Действие | Description |
+| ----------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `отключить` | Triggered when an organization owner disables the dependency graph for all existing repositories. For more information, see "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)." |
+| `включить` | Triggered when an organization owner enables the dependency graph for all existing repositories. |
+
+#### `dependency_graph_new_repos` category actions
+
+| Действие | Description |
+| ----------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `отключить` | Triggered when an organization owner disables the dependency graph for all new repositories. For more information, see "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)." |
+| `включить` | Triggered when an organization owner enables the dependency graph for all new repositories. |
+
{% endif %}
-##### The `discussion_post` category
+#### `discussion_post` category actions
| Действие | Description |
| ------------ | --------------------------------------------------------------------------------------------------------------- |
| `обновление` | Triggered when [a team discussion post is edited](/articles/managing-disruptive-comments/#editing-a-comment). |
| `destroy` | Triggered when [a team discussion post is deleted](/articles/managing-disruptive-comments/#deleting-a-comment). |
-##### The `discussion_post_reply` category
+#### `discussion_post_reply` category actions
| Действие | Description |
| ------------ | -------------------------------------------------------------------------------------------------------------------------- |
| `обновление` | Triggered when [a reply to a team discussion post is edited](/articles/managing-disruptive-comments/#editing-a-comment). |
| `destroy` | Triggered when [a reply to a team discussion post is deleted](/articles/managing-disruptive-comments/#deleting-a-comment). |
-##### The `hook` category
+#### `hook` category actions
| Действие | Description |
| ---------------- | -------------------------------------------------------------------------------------------------------------- |
@@ -133,14 +223,14 @@ This list describes the available categories and associated events:
| `destroy` | Triggered when an existing hook was removed from a repository. |
| `events_changed` | Triggered when the events on a hook have been altered. |
-##### The `integration_installation_request` category
+#### `integration_installation_request` category actions
| Действие | Description |
| -------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| `create` | Triggered when an organization member requests that an organization owner install an integration for use in the organization. |
| `close` | Triggered when a request to install an integration for use in an organization is either approved or denied by an organization owner, or canceled by the organization member who opened the request. |
-##### The `issue` category
+#### `issue` category actions
| Действие | Description |
| --------- | ---------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -148,13 +238,13 @@ This list describes the available categories and associated events:
{% if currentVersion == "free-pro-team@latest" %}
-##### The `marketplace_agreement_signature` category
+#### `marketplace_agreement_signature` category actions
| Действие | Description |
| -------- | -------------------------------------------------------------------------------------------------- |
| `create` | Triggered when you sign the {% data variables.product.prodname_marketplace %} Developer Agreement. |
-##### The `marketplace_listing` category
+#### `marketplace_listing` category actions
| Действие | Description |
| ----------- | --------------------------------------------------------------------------------------------------------------- |
@@ -168,7 +258,7 @@ This list describes the available categories and associated events:
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
-##### The `members_can_create_pages` category
+#### `members_can_create_pages` category actions
For more information, see "[Restricting publication of {% data variables.product.prodname_pages %} sites for your organization](/github/setting-up-and-managing-organizations-and-teams/disabling-publication-of-github-pages-sites-for-your-organization)."
@@ -179,7 +269,7 @@ For more information, see "[Restricting publication of {% data variables.product
{% endif %}
-##### The `org` category
+#### `org` category actions
| Действие | Description |
| -------------------------------------------------------------------------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |{% if currentVersion == "free-pro-team@latest"%}
@@ -222,7 +312,7 @@ For more information, see "[Restricting publication of {% data variables.product
| `update_terms_of_service` | Triggered when an organization changes between the Standard Terms of Service and the Corporate Terms of Service. For more information, see "[Upgrading to the Corporate Terms of Service](/articles/upgrading-to-the-corporate-terms-of-service)."{% endif %}
{% if currentVersion == "free-pro-team@latest" %}
-##### The `org_credential_authorization` category
+#### `org_credential_authorization` category actions
| Действие | Description |
| -------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -233,7 +323,7 @@ For more information, see "[Restricting publication of {% data variables.product
{% endif %}
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "github-ae@latest" %}
-##### The `organization_label` category
+#### `organization_label` category actions
| Действие | Description |
| ------------ | ------------------------------------------ |
@@ -243,7 +333,7 @@ For more information, see "[Restricting publication of {% data variables.product
{% endif %}
-##### The `oauth_application` category
+#### `oauth_application` category actions
| Действие | Description |
| --------------- | ------------------------------------------------------------------------------------------------------------------ |
@@ -255,7 +345,7 @@ For more information, see "[Restricting publication of {% data variables.product
{% if currentVersion == "free-pro-team@latest" %}
-##### The `payment_method` category
+#### `payment_method` category actions
| Действие | Description |
| ------------ | ------------------------------------------------------------------------------------------ |
@@ -265,12 +355,12 @@ For more information, see "[Restricting publication of {% data variables.product
{% endif %}
-##### The `profile_picture` category
+#### `profile_picture` category actions
| Действие | Description |
| ---------- | --------------------------------------------------------------------- |
| обновление | Triggered when you set or update your organization's profile picture. |
-##### The `project` category
+#### `project` category actions
| Действие | Description |
| ------------------------ | ------------------------------------------------------------------------------------------------------------------------------------------------ |
@@ -284,7 +374,7 @@ For more information, see "[Restricting publication of {% data variables.product
| `update_team_permission` | Triggered when a team's project board permission level is changed or when a team is added or removed from a project board. |
| `update_user_permission` | Triggered when an organization member or outside collaborator is added to or removed from a project board or has their permission level changed. |
-##### The `protected_branch` category
+#### `protected_branch` category actions
| Действие | Description |
| ----------------------------------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -304,14 +394,14 @@ For more information, see "[Restricting publication of {% data variables.product
| `update_linear_history_requirement_enforcement_level` | Triggered when required linear commit history is enabled or disabled for a protected branch. |
{% endif %}
-##### The `repo` category
+#### `repo` category actions
| Действие | Description |
| ------------------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `access` | Triggered when a repository owned by an organization is [switched from "private" to "public"](/articles/making-a-private-repository-public) (or vice versa). |
+| `access` | Triggered when a user [changes the visibility](/github/administering-a-repository/setting-repository-visibility) of a repository in the organization. |
| `add_member` | Triggered when a user accepts an [invitation to have collaboration access to a repository](/articles/inviting-collaborators-to-a-personal-repository). |
| `add_topic` | Triggered when a repository admin [adds a topic](/articles/classifying-your-repository-with-topics) to a repository. |
-| `archived` | Triggered when a repository admin [archives a repository](/articles/about-archiving-repositories).{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
+| `archived` | Triggered when a repository admin [archives a repository](/articles/about-archiving-repositories).{% if enterpriseServerVersions contains currentVersion %}
| `config.disable_anonymous_git_access` | Triggered when [anonymous Git read access is disabled](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository) in a public repository. |
| `config.enable_anonymous_git_access` | Triggered when [anonymous Git read access is enabled](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository) in a public repository. |
| `config.lock_anonymous_git_access` | Triggered when a repository's [anonymous Git read access setting is locked](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access). |
@@ -334,35 +424,80 @@ For more information, see "[Restricting publication of {% data variables.product
{% if currentVersion == "free-pro-team@latest" %}
-##### The `repository_content_analysis` category
+#### `repository_advisory` category actions
+
+| Действие | Description |
+| ------------------ | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| `close` | Triggered when someone closes a security advisory. For more information, see "[About {% data variables.product.prodname_dotcom %} Security Advisories](/github/managing-security-vulnerabilities/about-github-security-advisories)." |
+| `cve_request` | Triggered when someone requests a CVE (Common Vulnerabilities and Exposures) number from {% data.variables.product.prodname_dotcom %} for a draft security advisory. |
+| `github_broadcast` | Triggered when {% data.variables.product.prodname_dotcom %} makes a security advisory public in the {% data variables.product.prodname_advisory_database %}. |
+| `github_withdraw` | Triggered when {% data.variables.product.prodname_dotcom %} withdraws a security advisory that was published in error. |
+| `open` | Triggered when someone opens a draft security advisory. |
+| `publish` | Triggered when someone publishes a security advisory. |
+| `reopen` | Triggered when someone reopens as draft security advisory. |
+| `обновление` | Triggered when someone edits a draft or published security advisory. |
+
+#### `repository_content_analysis` category actions
| Действие | Description |
| ----------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| `включить` | Triggered when an organization owner or person with admin access to the repository [enables data use settings for a private repository](/github/understanding-how-github-uses-and-protects-your-data/managing-data-use-settings-for-your-private-repository). |
| `отключить` | Triggered when an organization owner or person with admin access to the repository [disables data use settings for a private repository](/github/understanding-how-github-uses-and-protects-your-data/managing-data-use-settings-for-your-private-repository). |
-##### The `repository_dependency_graph` category
+{% endif %}{% if currentVersion != "github-ae@latest" %}
-| Действие | Description |
-| ----------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `включить` | Triggered when a repository owner or person with admin access to the repository [enables the dependency graph for a private repository](/github/visualizing-repository-data-with-graphs/exploring-the-dependencies-and-dependents-of-a-repository). |
-| `отключить` | Triggered when a repository owner or person with admin access to the repository [disables the dependency graph for a private repository](/github/visualizing-repository-data-with-graphs/exploring-the-dependencies-and-dependents-of-a-repository). |
+#### `repository_dependency_graph` category actions
+
+| Действие | Description |
+| ----------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `отключить` | Triggered when a repository owner or person with admin access to the repository disables the dependency graph for a {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repository. For more information, see "[About the dependency graph](/github/visualizing-repository-data-with-graphs/about-the-dependency-graph)." |
+| `включить` | Triggered when a repository owner or person with admin access to the repository enables the dependency graph for a {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repository. |
+
+{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %}
+#### `repository_secret_scanning` category actions
+
+| Действие | Description |
+| ----------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `отключить` | Triggered when a repository owner or person with admin access to the repository disables secret scanning for a {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repository. For more information, see "[About secret scanning](/github/administering-a-repository/about-secret-scanning)." |
+| `включить` | Triggered when a repository owner or person with admin access to the repository enables secret scanning for a {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repository. |
+
+{% endif %}{% if currentVersion != "github-ae@latest" %}
+#### `repository_vulnerability_alert` category actions
+
+| Действие | Description |
+| -------------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `create` | Triggered when {% data variables.product.product_name %} creates a {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot %}{% else %}security{% endif %} alert for a repository that uses a vulnerable dependency. For more information, see "[About alerts for vulnerable dependencies](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies)." |
+| `отклонить` | Triggered when an organization owner or person with admin access to the repository dismisses a {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot %}{% else %}security{% endif %} alert about a vulnerable dependency. |
+| `разрешение проблем` | Triggered when someone with write access to a repository pushes changes to update and resolve a vulnerability in a project dependency. |
+
+{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+#### `repository_vulnerability_alerts` category actions
+
+| Действие | Description |
+| ------------------------ | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| `authorized_users_teams` | Triggered when an organization owner or a person with admin permissions to the repository updates the list of people or teams authorized to receive {% data variables.product.prodname_dependabot_alerts %} for vulnerable dependencies in the repository. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-security-alerts)." |
+| `отключить` | Triggered when a repository owner or person with admin access to the repository disables {% data variables.product.prodname_dependabot_alerts %}. |
+| `включить` | Triggered when a repository owner or person with admin access to the repository enables {% data variables.product.prodname_dependabot_alerts %}. |
+
+{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %}
+#### `secret_scanning` category actions
+
+| Действие | Description |
+| ----------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `отключить` | Triggered when an organization owner disables secret scanning for all existing{% if currentVersion == "free-pro-team@latest" %}, private{% endif %} repositories. For more information, see "[About secret scanning](/github/administering-a-repository/about-secret-scanning)." |
+| `включить` | Triggered when an organization owner enables secret scanning for all existing{% if currentVersion == "free-pro-team@latest" %}, private{% endif %} repositories. |
+
+#### `secret_scanning_new_repos` category actions
+
+| Действие | Description |
+| ----------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `отключить` | Triggered when an organization owner disables secret scanning for all new {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repositories. For more information, see "[About secret scanning](/github/administering-a-repository/about-secret-scanning)." |
+| `включить` | Triggered when an organization owner enables secret scanning for all new {% if currentVersion == "free-pro-team@latest" %}private {% endif %}repositories. |
-{% endif %}
-{% if currentVersion != "github-ae@latest" %}
-##### The `repository_vulnerability_alert` category
-
-| Действие | Description |
-| ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `create` | Triggered when {% data variables.product.product_name %} creates a [{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot %}{% else %}security{% endif %} alert for a vulnerable dependency](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies) in a particular repository. |
-| `разрешение проблем` | Triggered when someone with write access to a repository [pushes changes to update and resolve a vulnerability](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies) in a project dependency. |
-| `отклонить` | Triggered when an organization owner or person with admin access to the repository dismisses a |
-| {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot %}{% else %}security{% endif %} alert about a vulnerable dependency.{% if currentVersion == "free-pro-team@latest" %} | |
-| `authorized_users_teams` | Triggered when an organization owner or a member with admin permissions to the repository [updates the list of people or teams authorized to receive {% data variables.product.prodname_dependabot_alerts %}](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-dependabot-alerts) for vulnerable dependencies in the repository.{% endif %}
{% endif %}
{% if currentVersion == "free-pro-team@latest" %}
-##### The `sponsors` category
+#### `sponsors` category actions
| Действие | Description |
| ----------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -371,7 +506,7 @@ For more information, see "[Restricting publication of {% data variables.product
{% endif %}
{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-##### The `team` category
+#### `team` category actions
| Действие | Description |
| -------------------- | --------------------------------------------------------------------------------------------------------------------------------------- |
@@ -385,60 +520,13 @@ For more information, see "[Restricting publication of {% data variables.product
| `remove_repository` | Triggered when a repository is no longer under a team's control. |
{% endif %}
-##### The `team_discussions` category
+#### `team_discussions` category actions
| Действие | Description |
| ----------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| `отключить` | Triggered when an organization owner disables team discussions for an organization. For more information, see "[Disabling team discussions for your organization](/articles/disabling-team-discussions-for-your-organization)." |
| `включить` | Triggered when an organization owner enables team discussions for an organization. |
-#### Search based on time of action
-
-Use the `created` qualifier to filter actions in the audit log based on when they occurred. {% data reusables.time_date.date_format %} {% data reusables.time_date.time_format %}
-
-{% data reusables.search.date_gt_lt %} For example:
-
- * `created:2014-07-08` finds all events that occurred on July 8th, 2014.
- * `created:>=2014-07-08` finds all events that occurred on or after July 8th, 2014.
- * `created:<=2014-07-08` finds all events that occurred on or before July 8th, 2014.
- * `created:2014-07-01..2014-07-31` finds all events that occurred in the month of July 2014.
-
-The audit log contains data for the past 90 days, but you can use the `created` qualifier to search for events earlier than that.
-
-#### Search based on location
-
-Using the qualifier `country`, you can filter actions in the audit log based on the originating country. You can use a country's two-letter short code or its full name. Keep in mind that countries with spaces in their name will need to be wrapped in quotation marks. Например:
-
- * `country:de` finds all events that occurred in Germany.
- * `country:Mexico` finds all events that occurred in Mexico.
- * `country:"United States"` all finds events that occurred in the United States.
-
-{% if currentVersion == "free-pro-team@latest" %}
-### Exporting the audit log
-
-{% data reusables.audit_log.export-log %}
-{% data reusables.audit_log.exported-log-keys-and-values %}
-{% endif %}
-
-### Using the Audit log API
-
-{% note %}
-
-**Note**: The Audit log API is available for organizations using {% data variables.product.prodname_enterprise %}. {% data reusables.gated-features.more-info-org-products %}
-
-{% endnote %}
-
-To ensure a secure IP and maintain compliance for your organization, you can use the Audit log API to keep copies of your audit log data and monitor:
-* Access to your organization or repository settings.
-* Changes in permissions.
-* Added or removed users in an organization, repository, or team.
-* Users being promoted to admin.
-* Changes to permissions of a GitHub App.
-
-The GraphQL response can include data for up to 90 to 120 days.
-
-For example, you can make a GraphQL request to see all the new organization members added to your organization. For more information, see the "[GraphQL API Audit Log](/v4/interface/auditentry/)."
-
### Дополнительная литература
- "[Keeping your organization secure](/articles/keeping-your-organization-secure)"
diff --git a/translations/ru-RU/content/github/setting-up-and-managing-your-enterprise/about-enterprise-accounts.md b/translations/ru-RU/content/github/setting-up-and-managing-your-enterprise/about-enterprise-accounts.md
index bedbb2ace51b..ea38298892b7 100644
--- a/translations/ru-RU/content/github/setting-up-and-managing-your-enterprise/about-enterprise-accounts.md
+++ b/translations/ru-RU/content/github/setting-up-and-managing-your-enterprise/about-enterprise-accounts.md
@@ -20,7 +20,7 @@ An enterprise account allows you to manage multiple {% data variables.product.pr
- Security (single sign-on, two factor authentication)
- Requests and support bundle sharing with {% data variables.contact.enterprise_support %}
-{% data reusables.enterprise-accounts.enterprise-accounts-billing %}
+{% data reusables.enterprise-accounts.enterprise-accounts-billing %} For more information about managing your {% data variables.product.prodname_ghe_cloud %} subscription, see "[Viewing the subscription and usage for your enterprise account](/articles/viewing-the-subscription-and-usage-for-your-enterprise-account)." For more information about managing your {% data variables.product.prodname_ghe_server %} billing settings, see "[Managing billing for your enterprise](/admin/overview/managing-billing-for-your-enterprise)."
For more information about the differences between {% data variables.product.prodname_ghe_cloud %} and {% data variables.product.prodname_ghe_server %}, see "[{% data variables.product.prodname_dotcom %}'s products](/articles/githubs-products)." To upgrade to {% data variables.product.prodname_enterprise %} or to get started with an enterprise account, contact {% data variables.contact.contact_enterprise_sales %}.
diff --git a/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/about-your-profile.md b/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/about-your-profile.md
index b472d23b0ae1..cbb5b65177bf 100644
--- a/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/about-your-profile.md
+++ b/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/about-your-profile.md
@@ -13,7 +13,7 @@ versions:
You can add personal information about yourself in your bio, like previous places you've worked, projects you've contributed to, or interests you have that other people may like to know about. For more information, see "[Adding a bio to your profile](/articles/personalizing-your-profile/#adding-a-bio-to-your-profile)."
-{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
{% data reusables.profile.profile-readme %}
@@ -23,19 +23,17 @@ You can add personal information about yourself in your bio, like previous place
People who visit your profile see a timeline of your contribution activity, like issues and pull requests you've opened, commits you've made, and pull requests you've reviewed. You can choose to display only public contributions or to also include private, anonymized contributions. For more information, see "[Viewing contributions on your profile page](/articles/viewing-contributions-on-your-profile-page)" or "[Publicizing or hiding your private contributions on your profile](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)."
-They can also see:
+People who visit your profile can also see the following information.
-- Repositories and gists you own or contribute to. You can showcase your best work by pinning repositories and gists to your profile. For more information, see "[Pinning items to your profile](/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile)."
-- Repositories you've starred. For more information, see "[Saving repositories with stars](/articles/saving-repositories-with-stars/)"
-- An overview of your activity in organizations, repositories, and teams you're most active in. For more information, see "[Showing an overview of your activity on your profile](/articles/showing-an-overview-of-your-activity-on-your-profile).{% if currentVersion == "free-pro-team@latest" %}
-- Badges that advertise your participation in programs like the {% data variables.product.prodname_arctic_vault %}, {% data variables.product.prodname_sponsors %}, or the {% data variables.product.company_short %} Developer Program.
-- If you're using {% data variables.product.prodname_pro %}. For more information, see "[Personalizing your profile](/articles/personalizing-your-profile)."{% endif %}
+- Repositories and gists you own or contribute to. {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}You can showcase your best work by pinning repositories and gists to your profile. For more information, see "[Pinning items to your profile](/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile)."{% endif %}
+- Repositories you've starred. For more information, see "[Saving repositories with stars](/articles/saving-repositories-with-stars/)."
+- An overview of your activity in organizations, repositories, and teams you're most active in. For more information, see "[Showing an overview of your activity on your profile](/articles/showing-an-overview-of-your-activity-on-your-profile)."{% if currentVersion == "free-pro-team@latest" %}
+- Badges that show if you use {% data variables.product.prodname_pro %} or participate in programs like the {% data variables.product.prodname_arctic_vault %}, {% data variables.product.prodname_sponsors %}, or the {% data variables.product.company_short %} Developer Program. For more information, see "[Personalizing your profile](/github/setting-up-and-managing-your-github-profile/personalizing-your-profile#displaying-badges-on-your-profile)."{% endif %}
You can also set a status on your profile to provide information about your availability. For more information, see "[Setting a status](/articles/personalizing-your-profile/#setting-a-status)."
### Дополнительная литература
- "[How do I set up my profile picture?](/articles/how-do-i-set-up-my-profile-picture)"
-- "[Pinning repositories to your profile](/articles/pinning-repositories-to-your-profile)"
- "[Publicizing or hiding your private contributions on your profile](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)"
- "[Viewing contributions on your profile](/articles/viewing-contributions-on-your-profile)"
diff --git a/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme.md b/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme.md
index 3f8c1cd88927..89bf76b52c11 100644
--- a/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme.md
+++ b/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme.md
@@ -4,7 +4,6 @@ intro: 'You can add a README to your {% data variables.product.prodname_dotcom %
versions:
free-pro-team: '*'
enterprise-server: '>=2.22'
- github-ae: '*'
---
### About your profile README
diff --git a/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/personalizing-your-profile.md b/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/personalizing-your-profile.md
index 8995645f6b09..626961bb4fca 100644
--- a/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/personalizing-your-profile.md
+++ b/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/personalizing-your-profile.md
@@ -50,9 +50,9 @@ You can change the name that is displayed on your profile. This name may also be
Add a bio to your profile to share information about yourself with other {% data variables.product.product_name %} users. With the help of [@mentions](/articles/basic-writing-and-formatting-syntax) and emoji, you can include information about where you currently or have previously worked, what type of work you do, or even what kind of coffee you drink.
-{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
-For a longer-form and more prominent way of displaying customized information about yourself, you can also use a profile README. For more information on the profile README, see "[Managing your profile README](/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme)."
+For a longer-form and more prominent way of displaying customized information about yourself, you can also use a profile README. For more information, see "[Managing your profile README](/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme)."
{% endif %}
diff --git a/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile.md b/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile.md
index 35c99575cc50..1d5f98c4fc11 100644
--- a/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile.md
+++ b/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile.md
@@ -7,7 +7,6 @@ redirect_from:
versions:
free-pro-team: '*'
enterprise-server: '*'
- github-ae: '*'
---
You can pin a public repository if you own the repository or you've made contributions to the repository. Commits to forks don't count as contributions, so you can't pin a fork that you don't own. For more information, see "[Why are my contributions not showing up on my profile?](/articles/why-are-my-contributions-not-showing-up-on-my-profile)"
diff --git a/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/publicizing-or-hiding-your-private-contributions-on-your-profile.md b/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/publicizing-or-hiding-your-private-contributions-on-your-profile.md
index 669494909eab..0575f346467a 100644
--- a/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/publicizing-or-hiding-your-private-contributions-on-your-profile.md
+++ b/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/publicizing-or-hiding-your-private-contributions-on-your-profile.md
@@ -1,6 +1,6 @@
---
title: Publicizing or hiding your private contributions on your profile
-intro: 'Your {% data variables.product.product_name %} profile shows a graph of your repository contributions over the past year. You can choose to show anonymized activity from private repositories in addition to the activity shown from public repositories.'
+intro: 'Your {% data variables.product.product_name %} profile shows a graph of your repository contributions over the past year. You can choose to show anonymized activity from {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" %}private and internal{% else %}private{% endif %} repositories{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %} in addition to the activity from public repositories{% endif %}.'
redirect_from:
- /articles/publicizing-or-hiding-your-private-contributions-on-your-profile
versions:
diff --git a/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/viewing-contributions-on-your-profile.md b/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/viewing-contributions-on-your-profile.md
index 1dca83a8b60e..7a366d5cccc8 100644
--- a/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/viewing-contributions-on-your-profile.md
+++ b/translations/ru-RU/content/github/setting-up-and-managing-your-github-profile/viewing-contributions-on-your-profile.md
@@ -1,6 +1,6 @@
---
title: Viewing contributions on your profile
-intro: 'Your {% data variables.product.product_name %} profile shows off your pinned repositories as well as a graph of your repository contributions over the past year.'
+intro: 'Your {% data variables.product.product_name %} profile shows off {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}your pinned repositories as well as{% endif %} a graph of your repository contributions over the past year.'
redirect_from:
- /articles/viewing-contributions/
- /articles/viewing-contributions-on-your-profile-page/
@@ -11,7 +11,7 @@ versions:
github-ae: '*'
---
-Your contribution graph shows activity from public repositories. You can choose to show activity from both public and private repositories, with specific details of your activity in private repositories anonymized. For more information, see "[Publicizing or hiding your private contributions on your profile](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)."
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}Your contribution graph shows activity from public repositories. {% endif %}You can choose to show activity from {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}both public and{% endif %}private repositories, with specific details of your activity in private repositories anonymized. For more information, see "[Publicizing or hiding your private contributions on your profile](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)."
{% note %}
@@ -33,16 +33,20 @@ On your profile page, certain actions count as contributions:
### Popular repositories
-This section displays your repositories with the most watchers. Once you [pin repositories to your profile](/articles/pinning-repositories-to-your-profile), this section will change to "Pinned repositories."
+This section displays your repositories with the most watchers. {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}Once you [pin repositories to your profile](/articles/pinning-repositories-to-your-profile), this section will change to "Pinned repositories."{% endif %}

+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
### Pinned repositories
This section displays up to six public repositories and can include your repositories as well as repositories you've contributed to. To easily see important details about the repositories you've chosen to feature, each repository in this section includes a summary of the work being done, the number of [stars](/articles/saving-repositories-with-stars/) the repository has received, and the main programming language used in the repository. For more information, see "[Pinning repositories to your profile](/articles/pinning-repositories-to-your-profile)."

+{% endif %}
+
### Contributions calendar
Your contributions calendar shows your contribution activity.
diff --git a/translations/ru-RU/content/github/setting-up-and-managing-your-github-user-account/about-your-personal-dashboard.md b/translations/ru-RU/content/github/setting-up-and-managing-your-github-user-account/about-your-personal-dashboard.md
index a707716509e5..effcfd2b9e1c 100644
--- a/translations/ru-RU/content/github/setting-up-and-managing-your-github-user-account/about-your-personal-dashboard.md
+++ b/translations/ru-RU/content/github/setting-up-and-managing-your-github-user-account/about-your-personal-dashboard.md
@@ -15,7 +15,7 @@ versions:
Your personal dashboard is the first page you'll see when you sign in on {% data variables.product.product_name %}.
-To access your personal dashboard once you're signed in, click the {% octicon "mark-github" aria-label="The github octocat logo" %} in the upper-left corner of any page on {% data variables.product.product_url %}.
+To access your personal dashboard once you're signed in, click the {% octicon "mark-github" aria-label="The github octocat logo" %} in the upper-left corner of any page on {% data variables.product.product_name %}.
### Finding your recent activity
@@ -39,11 +39,11 @@ In the "All activity" section of your news feed, you can view updates from repos
You'll see updates in your news feed when a user you follow:
- Stars a repository.
-- Follows another user.
-- Creates a public repository.
+- Follows another user.{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- Creates a public repository.{% endif %}
- Opens an issue or pull request with "help wanted" or "good first issue" label on a repository you're watching.
-- Pushes commits to a repository you watch.
-- Forks a public repository.
+- Pushes commits to a repository you watch.{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- Forks a public repository.{% endif %}
For more information about starring repositories and following people, see "[Saving repositories with stars](/articles/saving-repositories-with-stars/)" and "[Following people](/articles/following-people)."
diff --git a/translations/ru-RU/content/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository.md b/translations/ru-RU/content/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository.md
index 926e5594efb0..46cf747ac800 100644
--- a/translations/ru-RU/content/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository.md
+++ b/translations/ru-RU/content/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository.md
@@ -50,7 +50,7 @@ Repositories owned by an organization can grant more granular access. For more i
### Дополнительная литература
-- "[Permission levels for a user account repository](/articles/permission-levels-for-a-user-account-repository/#collaborator-access-on-a-repository-owned-by-a-user-account)"
+- "[Permission levels for a user account repository](/articles/permission-levels-for-a-user-account-repository/#collaborator-access-for-a-repository-owned-by-a-user-account)"
- "[Removing a collaborator from a personal repository](/articles/removing-a-collaborator-from-a-personal-repository)"
- "[Removing yourself from a collaborator's repository](/articles/removing-yourself-from-a-collaborator-s-repository)"
- "[Organizing members into teams](/articles/organizing-members-into-teams)"
diff --git a/translations/ru-RU/content/github/setting-up-and-managing-your-github-user-account/permission-levels-for-a-user-account-repository.md b/translations/ru-RU/content/github/setting-up-and-managing-your-github-user-account/permission-levels-for-a-user-account-repository.md
index 442bc4111d78..010c18a45076 100644
--- a/translations/ru-RU/content/github/setting-up-and-managing-your-github-user-account/permission-levels-for-a-user-account-repository.md
+++ b/translations/ru-RU/content/github/setting-up-and-managing-your-github-user-account/permission-levels-for-a-user-account-repository.md
@@ -1,6 +1,6 @@
---
title: Permission levels for a user account repository
-intro: 'A repository owned by a user account has two permission levels: the *repository owner* and *collaborators*.'
+intro: 'A repository owned by a user account has two permission levels: the repository owner and collaborators.'
redirect_from:
- /articles/permission-levels-for-a-user-account-repository
versions:
@@ -9,38 +9,48 @@ versions:
github-ae: '*'
---
+### About permissions levels for a user account repository
+
+Repositories owned by user accounts have one owner. Ownership permissions can't be shared with another user account.
+
+You can also {% if currentVersion == "free-pro-team@latest" %}invite{% else %}add{% endif %} users on {% data variables.product.product_name %} to your repository as collaborators. For more information, see "[Inviting collaborators to a personal repository](/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository)."
+
{% tip %}
-**Tip:** If you require more granular read/write access to a repository owned by your user account, consider transferring the repository to an organization. For more information, see "[Transferring a repository](/articles/transferring-a-repository)."
+**Tip:** If you require more granular access to a repository owned by your user account, consider transferring the repository to an organization. For more information, see "[Transferring a repository](/github/administering-a-repository/transferring-a-repository#transferring-a-repository-owned-by-your-user-account)."
{% endtip %}
-#### Owner access on a repository owned by a user account
-
-The repository owner has full control of the repository. In addition to all the permissions allowed by repository collaborators, the repository owner can:
-
-- {% if currentVersion == "free-pro-team@latest" %}[Invite collaborators](/articles/inviting-collaborators-to-a-personal-repository){% else %}[Add collaborators](/articles/inviting-collaborators-to-a-personal-repository){% endif %}
-- Change the visibility of the repository (from [public to private](/articles/making-a-public-repository-private), or from [private to public](/articles/making-a-private-repository-public)){% if currentVersion == "free-pro-team@latest" %}
-- [Limit interactions with a repository](/articles/limiting-interactions-with-your-repository){% endif %}
-- Merge a pull request on a protected branch, even if there are no approving reviews
-- [Delete the repository](/articles/deleting-a-repository)
-- [Manage a repository's topics](/articles/classifying-your-repository-with-topics){% if currentVersion == "free-pro-team@latest" %}
-- Manage security and analysis settings. For more information, see "[Managing security and analysis settings for your user account](/github/setting-up-and-managing-your-github-user-account/managing-security-and-analysis-settings-for-your-user-account)."{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-- [Enable the dependency graph](/github/visualizing-repository-data-with-graphs/exploring-the-dependencies-and-dependents-of-a-repository) for a private repository{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-- Delete packages. For more information, see "[Deleting a package](/github/managing-packages-with-github-packages/deleting-a-package)."{% endif %}
-- Create and edit repository social cards. For more information, see "[Customizing your repository's social media preview](/articles/customizing-your-repositorys-social-media-preview)."
-- Make the repository a template. For more information, see "[Creating a template repository](/articles/creating-a-template-repository)."{% if currentVersion != "github-ae@latest" %}
-- Receive [{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot_alerts %}{% else %}security alerts{% endif %} for vulnerable dependencies](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies) in a repository.{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-- Dismiss {% data variables.product.prodname_dependabot_alerts %} in your repository. For more information, see "[Viewing and updating vulnerable dependencies in your repository](/github/managing-security-vulnerabilities/viewing-and-updating-vulnerable-dependencies-in-your-repository)."
-- [Manage data usage for your private repository](/github/understanding-how-github-uses-and-protects-your-data/managing-data-use-settings-for-your-private-repository){% endif %}
-- [Define code owners for the repository](/articles/about-code-owners)
-- [Archive repositories](/articles/about-archiving-repositories){% if currentVersion == "free-pro-team@latest" %}
-- Create security advisories. For more information, see "[About {% data variables.product.prodname_security_advisories %}](/github/managing-security-vulnerabilities/about-github-security-advisories)."
-- Display a sponsor button. For more information, see "[Displaying a sponsor button in your repository](/articles/displaying-a-sponsor-button-in-your-repository)."{% endif %}
-
-There is only **one owner** of a repository owned by a user account; this permission cannot be shared with another user account. To transfer ownership of a repository to another user, see "[How to transfer a repository](/articles/how-to-transfer-a-repository)."
-
-#### Collaborator access on a repository owned by a user account
+### Owner access for a repository owned by a user account
+
+The repository owner has full control of the repository. In addition to the actions that any collaborator can perform, the repository owner can perform the following actions.
+
+| Действие | More information |
+|:---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |:----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| {% if currentVersion == "free-pro-team@latest" %}Invite collaborators{% else %}Add collaborators{% endif %} | |
+| "[Inviting collaborators to a personal repository](/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository)" | |
+| Change the visibility of the repository | "[Setting repository visibility](/github/administering-a-repository/setting-repository-visibility)" |{% if currentVersion == "free-pro-team@latest" %}
+| Limit interactions with the repository | "[Limiting interactions in your repository](/github/building-a-strong-community/limiting-interactions-in-your-repository)" |{% endif %}
+| Merge a pull request on a protected branch, even if there are no approving reviews | "[About protected branches](/github/administering-a-repository/about-protected-branches)" |
+| Delete the repository | "[Deleting a repository](/github/administering-a-repository/deleting-a-repository)" |
+| Manage the repository's topics | "[Classifying your repository with topics](/github/administering-a-repository/classifying-your-repository-with-topics)" |{% if currentVersion == "free-pro-team@latest" %}
+| Manage security and analysis settings for the repository | "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository)" |{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| Enable the dependency graph for a private repository | "[Exploring the dependencies of a repository](/github/visualizing-repository-data-with-graphs/exploring-the-dependencies-of-a-repository#enabling-and-disabling-the-dependency-graph-for-a-private-repository)" |{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| Delete packages | "[Deleting a package](/github/managing-packages-with-github-packages/deleting-a-package)" |{% endif %}
+| Customize the repository's social media preview | "[Customizing your repository's social media preview](/github/administering-a-repository/customizing-your-repositorys-social-media-preview)" |
+| Create a template from the repository | "[Creating a template repository](/github/creating-cloning-and-archiving-repositories/creating-a-template-repository)" |{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+| Receive | |
+| {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot_alerts %}{% else %}security alerts{% endif %} for vulnerable dependencies | "[About alerts for vulnerable dependencies](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies)" |{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| Dismiss {% data variables.product.prodname_dependabot_alerts %} in the repository | "[Viewing and updating vulnerable dependencies in your repository](/github/managing-security-vulnerabilities/viewing-and-updating-vulnerable-dependencies-in-your-repository)" |
+| Manage data use for a private repository | "[Managing data use settings for your private repository](/github/understanding-how-github-uses-and-protects-your-data/managing-data-use-settings-for-your-private-repository)"|{% endif %}
+| Define code owners for the repository | "[About code owners](/github/creating-cloning-and-archiving-repositories/about-code-owners)" |
+| Archive the repository | "[About archiving repositories](/github/creating-cloning-and-archiving-repositories/about-archiving-repositories)" |{% if currentVersion == "free-pro-team@latest" %}
+| Create security advisories | "[About {% data variables.product.prodname_security_advisories %}](/github/managing-security-vulnerabilities/about-github-security-advisories)" |
+| Display a sponsor button | "[Displaying a sponsor button in your repository](/github/administering-a-repository/displaying-a-sponsor-button-in-your-repository)" |{% endif %}
+
+### Collaborator access for a repository owned by a user account
+
+Collaborators on a personal repository can pull (read) the contents of the repository and push (write) changes to the repository.
{% note %}
@@ -48,27 +58,26 @@ There is only **one owner** of a repository owned by a user account; this permis
{% endnote %}
-Collaborators on a personal repository can:
-
-- Push to (write), pull from (read), and fork (copy) the repository
-- Create, apply, and delete labels and milestones
-- Open, close, re-open, and assign issues
-- Edit and delete comments on commits, pull requests, and issues
-- Mark an issue or pull request as a duplicate. For more information, see "[About duplicate issues and pull requests](/articles/about-duplicate-issues-and-pull-requests)."
-- Open, merge and close pull requests
-- Apply suggested changes to pull requests. For more information, see "[Incorporating feedback in your pull request](/articles/incorporating-feedback-in-your-pull-request)."
-- Send pull requests from forks of the repository{% if currentVersion == "free-pro-team@latest" %}
-- Publish, view, and install packages. For more information, see "[Publishing and managing packages](/github/managing-packages-with-github-packages/publishing-and-managing-packages)."{% endif %}
-- Create and edit Wikis
-- Create and edit releases. For more information, see "[Managing releases in a repository](/github/administering-a-repository/managing-releases-in-a-repository).
-- Remove themselves as collaborators on the repository
-- Submit a review on a pull request that will affect its mergeability
-- Act as a designated code owner for the repository. For more information, see "[About code owners](/articles/about-code-owners)."
-- Lock a conversation. For more information, see "[Locking conversations](/articles/locking-conversations)."{% if currentVersion == "free-pro-team@latest" %}
-- Report abusive content to {% data variables.contact.contact_support %}. For more information, see "[Reporting abuse or spam](/articles/reporting-abuse-or-spam)."{% endif %}
-- Transfer an issue to a different repository. For more information, see "[Transferring an issue to another repository](/articles/transferring-an-issue-to-another-repository)."
+Collaborators can also perform the following actions.
+
+| Действие | More information |
+|:----------------------------------------------------------------------------------------- |:------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| Fork the repository | "[About forks](/github/collaborating-with-issues-and-pull-requests/about-forks)" |
+| Create, edit, and delete comments on commits, pull requests, and issues in the repository | - "[About issues](/github/managing-your-work-on-github/about-issues)"
- "[Commenting on a pull request](/github/collaborating-with-issues-and-pull-requests/commenting-on-a-pull-request)"
- "[Managing disruptive comments](/github/building-a-strong-community/managing-disruptive-comments)"
|
+| Create, assign, close, and re-open issues in the repository | "[Managing your work with issues](/github/managing-your-work-on-github/managing-your-work-with-issues)" |
+| Manage labels for issues and pull requests in the repository | "[Labeling issues and pull requests](/github/managing-your-work-on-github/labeling-issues-and-pull-requests)" |
+| Manage milestones for issues and pull requests in the repository | "[Creating and editing milestones for issues and pull requests](/github/managing-your-work-on-github/creating-and-editing-milestones-for-issues-and-pull-requests)" |
+| Mark an issue or pull request in the repository as a duplicate | "[About duplicate issues and pull requests](/github/managing-your-work-on-github/about-duplicate-issues-and-pull-requests)" |
+| Create, merge, and close pull requests in the repository | "[Proposing changes to your work with pull requests](/github/collaborating-with-issues-and-pull-requests/proposing-changes-to-your-work-with-pull-requests)" |
+| Apply suggested changes to pull requests in the repository | "[Incorporating feedback in your pull request](/github/collaborating-with-issues-and-pull-requests/incorporating-feedback-in-your-pull-request)" |
+| Create a pull request from a fork of the repository | "[Creating a pull request from a fork](/github/collaborating-with-issues-and-pull-requests/creating-a-pull-request-from-a-fork)" |
+| Submit a review on a pull request that affects the mergeability of the pull request | "[Reviewing proposed changes in a pull request](/github/collaborating-with-issues-and-pull-requests/reviewing-proposed-changes-in-a-pull-request)" |
+| Create and edit a wiki for the repository | "[About wikis](/github/building-a-strong-community/about-wikis)" |
+| Create and edit releases for the repository | "[Managing releases in a repository](/github/administering-a-repository/managing-releases-in-a-repository)" |
+| Act as a code owner for the repository | "[About code owners](/articles/about-code-owners)" |{% if currentVersion == "free-pro-team@latest" %}
+| Publish, view, or install packages | "[Publishing and managing packages](/github/managing-packages-with-github-packages/publishing-and-managing-packages)" |{% endif %}
+| Remove themselves as collaborators on the repository | "[Removing yourself from a collaborator's repository](/github/setting-up-and-managing-your-github-user-account/removing-yourself-from-a-collaborators-repository)" |
### Дополнительная литература
-- "[Inviting collaborators to a personal repository](/articles/inviting-collaborators-to-a-personal-repository)"
- "[Repository permission levels for an organization](/articles/repository-permission-levels-for-an-organization)"
diff --git a/translations/ru-RU/content/github/site-policy/github-marketplace-terms-of-service.md b/translations/ru-RU/content/github/site-policy/github-marketplace-terms-of-service.md
index 036bc30e35a7..0d4dcedcec8d 100644
--- a/translations/ru-RU/content/github/site-policy/github-marketplace-terms-of-service.md
+++ b/translations/ru-RU/content/github/site-policy/github-marketplace-terms-of-service.md
@@ -6,11 +6,11 @@ versions:
free-pro-team: '*'
---
-Welcome to GitHub Marketplace ("Marketplace")! We're happy you're here. Please read these Terms of Service ("Marketplace Terms") carefully before accessing or using GitHub Marketplace. GitHub Marketplace is a platform that allows you to purchase developer products (for free or for a charge) that can be used with your GitHub.com account ("Developer Products"). Although sold by GitHub, Inc. ("GitHub", "we", "us"), Developer Products may be developed and maintained by either GitHub or by third-party software providers, and may require you to agree to a separate terms of service. Your use and/or purchase of Developer Products is subject to these Marketplace Terms and to the applicable fees and may also be subject to additional terms as provided by the third party licensor of that Developer Product (the "Product Provider").
+Welcome to GitHub Marketplace ("Marketplace")! We're happy you're here. Please read these Terms of Service ("Marketplace Terms") carefully before accessing or using GitHub Marketplace. GitHub Marketplace is a platform that allows you to select developer apps or actions (for free or for a charge) that can be used with your GitHub.com account ("Developer Products"). Although offered by GitHub, Inc. ("GitHub", "we", "us"), Developer Products may be developed and maintained by either GitHub or by third-party software providers. Your selection or use of Developer Products is subject to these Marketplace Terms and any applicable fees, and may require you to agree to additional terms as provided by the third party licensor of that Developer Product (the "Product Provider").
By using Marketplace, you are agreeing to be bound by these Marketplace Terms.
-Effective Date: October 11, 2017
+Effective Date: November 20, 2020
### A. GitHub.com's Terms of Service
@@ -40,11 +40,11 @@ If you would have a question, concern, or dispute regarding your billing, please
### E. Your Data and GitHub's Privacy Policy
-**Privacy.** When you purchase or subscribe to a Developer Product, GitHub must share certain Personal Information (as defined in the [GitHub Privacy Statement](/articles/github-privacy-statement/)) with the Product Provider in order to provide you with the Developer Product, regardless of your privacy settings. Depending on the requirements of the Developer Product you choose, GitHub may share as little as your user account name, ID, and primary email address or as much as access to the content in your repositories, including the ability to read and modify your private data. You will be able to view the scope of the permissions the Developer Product is requesting, and accept or deny them, when you grant it authorization via OAuth. In line with [GitHub's Privacy Statement](/articles/github-privacy-statement/), we will only provide the Product Provider with the minimum amount of information necessary for the purpose of the transaction.
+**Privacy.** When you select or use a Developer Product, GitHub may share certain Personal Information (as defined in the [GitHub Privacy Statement](/articles/github-privacy-statement/)) with the Product Provider (if any such Personal Information is received from you) in order to provide you with the Developer Product, regardless of your privacy settings. Depending on the requirements of the Developer Product you choose, GitHub may share as little as your user account name, ID, and primary email address or as much as access to the content in your repositories, including the ability to read and modify your private data. You will be able to view the scope of the permissions the Developer Product is requesting, and accept or deny them, when you grant it authorization via OAuth.
-If you cancel your Developer Product services and revoke access through your account settings, the Product Provider will no longer be able to access your account. The Product Provider is responsible for deleting your Personal Information from its systems within its defined window. Please contact the Product Provider to ensure that your account has been properly terminated.
+If you cease using the Developer Product and revoke access through your account settings, the Product Provider will no longer be able to access your account. The Product Provider is responsible for deleting your Personal Information from its systems within its defined window. Please contact the Product Provider to ensure that your account has been properly terminated.
-**Data Security Disclaimer.** When you purchase or subscribe to a Developer Product, the Developer Product security and their custodianship of your data is the responsibility of the Product Provider. You are responsible for understanding the security considerations of the purchase and use of the Developer Product for your own security, risk and compliance considerations.
+**Data Security and Privacy Disclaimer.** When you select or use a Developer Product, the security of the Developer Product and the custodianship of your data, including your Personal Information (if any), is the responsibility of the Product Provider. You are responsible for understanding the security and privacy considerations of the selection or use of the Developer Product for your own security and privacy risk and compliance considerations.
### F. Rights to Developer Products
diff --git a/translations/ru-RU/content/github/using-git/setting-your-username-in-git.md b/translations/ru-RU/content/github/using-git/setting-your-username-in-git.md
index bd543968d4ed..2652a54ea9f3 100644
--- a/translations/ru-RU/content/github/using-git/setting-your-username-in-git.md
+++ b/translations/ru-RU/content/github/using-git/setting-your-username-in-git.md
@@ -20,13 +20,13 @@ Changing the name associated with your Git commits using `git config` will only
2. {% data reusables.user_settings.set_your_git_username %}
```shell
$ git config --global user.name "Mona Lisa"
- ```
+ ```
3. {% data reusables.user_settings.confirm_git_username_correct %}
```shell
$ git config --global user.name
> Mona Lisa
- ```
+ ```
### Setting your Git username for a single repository
@@ -37,13 +37,13 @@ Changing the name associated with your Git commits using `git config` will only
3. {% data reusables.user_settings.set_your_git_username %}
```shell
$ git config user.name "Mona Lisa"
- ```
+ ```
3. {% data reusables.user_settings.confirm_git_username_correct %}
```shell
$ git config user.name
> Mona Lisa
- ```
+ ```
### Дополнительная литература
diff --git a/translations/ru-RU/content/github/using-git/which-remote-url-should-i-use.md b/translations/ru-RU/content/github/using-git/which-remote-url-should-i-use.md
index 170e478ac8a5..f6c233d9b05c 100644
--- a/translations/ru-RU/content/github/using-git/which-remote-url-should-i-use.md
+++ b/translations/ru-RU/content/github/using-git/which-remote-url-should-i-use.md
@@ -3,7 +3,7 @@ title: Which remote URL should I use?
redirect_from:
- /articles/which-url-should-i-use/
- /articles/which-remote-url-should-i-use
-intro: 'There are several ways to clone repositories available on {% data variables.product.prodname_dotcom %}.'
+intro: 'There are several ways to clone repositories available on {% data variables.product.product_location %}.'
versions:
free-pro-team: '*'
enterprise-server: '*'
@@ -16,7 +16,7 @@ For information on setting or changing your remote URL, see "[Changing a remote'
### Cloning with HTTPS URLs
-The `https://` clone URLs are available on all repositories, public and private. These URLs work even if you are behind a firewall or proxy.
+The `https://` clone URLs are available on all repositories, regardless of visibility. `https://` clone URLs work even if you are behind a firewall or proxy.
When you `git clone`, `git fetch`, `git pull`, or `git push` to a remote repository using HTTPS URLs on the command line, Git will ask for your {% data variables.product.product_name %} username and password. {% data reusables.user_settings.password-authentication-deprecation %}
diff --git a/translations/ru-RU/content/github/writing-on-github/creating-gists.md b/translations/ru-RU/content/github/writing-on-github/creating-gists.md
index 0f68b324b6ea..d5655b46bb08 100644
--- a/translations/ru-RU/content/github/writing-on-github/creating-gists.md
+++ b/translations/ru-RU/content/github/writing-on-github/creating-gists.md
@@ -33,8 +33,12 @@ You'll receive a notification when:
- Someone mentions you in a gist.
- You subscribe to a gist, by clicking **Subscribe** at the top any gist.
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
You can pin gists to your profile so other people can see them easily. For more information, see "[Pinning items to your profile](/articles/pinning-items-to-your-profile)."
+{% endif %}
+
You can discover gists others have created by going to the {% data variables.gists.gist_homepage %} and clicking **All Gists**. This will take you to a page of all gists sorted and displayed by time of creation or update. You can also search gists by language with {% data variables.gists.gist_search_url %}. Gist search uses the same search syntax as [code search](/articles/searching-code).
Since gists are Git repositories, you can view their full commit history, complete with diffs. You can also fork or clone gists. For more information, see ["Forking and cloning gists"](/articles/forking-and-cloning-gists).
diff --git a/translations/ru-RU/content/packages/managing-container-images-with-github-container-registry/deleting-a-container-image.md b/translations/ru-RU/content/packages/managing-container-images-with-github-container-registry/deleting-a-container-image.md
index ddf6eae62764..3fd9ac99bd21 100644
--- a/translations/ru-RU/content/packages/managing-container-images-with-github-container-registry/deleting-a-container-image.md
+++ b/translations/ru-RU/content/packages/managing-container-images-with-github-container-registry/deleting-a-container-image.md
@@ -16,10 +16,6 @@ To delete a container image, you must have admin permissions to the container im
When deleting public packages, be aware that you may break projects that depend on your package.
-### Reserved package versions and names
-
-{% data reusables.package_registry.package-immutability %}
-
### Deleting a version of a user-owned container image on {% data variables.product.prodname_dotcom %}
{% data reusables.package_registry.package-settings-from-user-level %}
diff --git a/translations/ru-RU/content/packages/publishing-and-managing-packages/deleting-a-package.md b/translations/ru-RU/content/packages/publishing-and-managing-packages/deleting-a-package.md
index dceaa32d12c9..7ce43daf8b0c 100644
--- a/translations/ru-RU/content/packages/publishing-and-managing-packages/deleting-a-package.md
+++ b/translations/ru-RU/content/packages/publishing-and-managing-packages/deleting-a-package.md
@@ -35,10 +35,6 @@ At this time, {% data variables.product.prodname_registry %} on {% data variable
{% endif %}
-### Reserved package versions and names
-
-{% data reusables.package_registry.package-immutability %}
-
### Deleting a version of a private package on {% data variables.product.product_name %}
To delete a private package version, you must have admin permissions in the repository.
diff --git a/translations/ru-RU/content/packages/publishing-and-managing-packages/publishing-a-package.md b/translations/ru-RU/content/packages/publishing-and-managing-packages/publishing-a-package.md
index d016cc2ba7c9..406c897528f5 100644
--- a/translations/ru-RU/content/packages/publishing-and-managing-packages/publishing-a-package.md
+++ b/translations/ru-RU/content/packages/publishing-and-managing-packages/publishing-a-package.md
@@ -18,8 +18,6 @@ You can help people understand and use your package by providing a description a
{% data reusables.package_registry.public-or-private-packages %} A repository can contain more than one package. To prevent confusion, make sure the README and description clearly provide information about each package.
-{% data reusables.package_registry.package-immutability %}
-
{% if currentVersion == "free-pro-team@latest" %}
If a new version of a package fixes a security vulnerability, you should publish a security advisory in your repository.
{% data variables.product.prodname_dotcom %} reviews each published security advisory and may use it to send {% data variables.product.prodname_dependabot_alerts %} to affected repositories. For more information, see "[About GitHub Security Advisories](/github/managing-security-vulnerabilities/about-github-security-advisories)."
diff --git a/translations/ru-RU/content/packages/using-github-packages-with-your-projects-ecosystem/configuring-npm-for-use-with-github-packages.md b/translations/ru-RU/content/packages/using-github-packages-with-your-projects-ecosystem/configuring-npm-for-use-with-github-packages.md
index 1c8b206dbb0d..f4320cce3b3b 100644
--- a/translations/ru-RU/content/packages/using-github-packages-with-your-projects-ecosystem/configuring-npm-for-use-with-github-packages.md
+++ b/translations/ru-RU/content/packages/using-github-packages-with-your-projects-ecosystem/configuring-npm-for-use-with-github-packages.md
@@ -45,12 +45,15 @@ If your instance has subdomain isolation disabled:
To authenticate by logging in to npm, use the `npm login` command, replacing *USERNAME* with your {% data variables.product.prodname_dotcom %} username, *TOKEN* with your personal access token, and *PUBLIC-EMAIL-ADDRESS* with your email address.
+If {% data variables.product.prodname_registry %} is not your default package registry for using npm and you want to use the `npm audit` command, we recommend you use the `--scope` flag with the owner of the package when you authenticate to {% data variables.product.prodname_registry %}.
+
{% if enterpriseServerVersions contains currentVersion %}
If your instance has subdomain isolation enabled:
{% endif %}
```shell
-$ npm login --registry=https://{% if currentVersion == "free-pro-team@latest" %}npm.pkg.github.com{% else %}npm.HOSTNAME/{% endif %}
+$ npm login --scope=@OWNER --registry=https://{% if currentVersion == "free-pro-team@latest" %}npm.pkg.github.com{% else %}npm.HOSTNAME/{% endif %}
+
> Username: USERNAME
> Password: TOKEN
> Email: PUBLIC-EMAIL-ADDRESS
@@ -60,7 +63,7 @@ $ npm login --registry=https://{% if currentVersion == "free-pro-team@latest" %}
If your instance has subdomain isolation disabled:
```shell
-$ npm login --registry=https://HOSTNAME/_registry/npm/
+$ npm login --scope=@OWNER --registry=https://HOSTNAME/_registry/npm/
> Username: USERNAME
> Password: TOKEN
> Email: PUBLIC-EMAIL-ADDRESS
diff --git a/translations/ru-RU/data/reusables/enterprise-accounts/enterprise-accounts-billing.md b/translations/ru-RU/data/reusables/enterprise-accounts/enterprise-accounts-billing.md
index cd4984777d60..1644a4007eaa 100644
--- a/translations/ru-RU/data/reusables/enterprise-accounts/enterprise-accounts-billing.md
+++ b/translations/ru-RU/data/reusables/enterprise-accounts/enterprise-accounts-billing.md
@@ -1 +1 @@
-Enterprise accounts are currently available to {% data variables.product.prodname_ghe_cloud %} and {% data variables.product.prodname_ghe_server %} customers paying by invoice. Billing for all of the organizations and {% data variables.product.prodname_ghe_server %} instances connected to your enterprise account is aggregated into a single bill. For more information about managing your {% data variables.product.prodname_ghe_cloud %} subscription, see "[Viewing the subscription and usage for your enterprise account](/articles/viewing-the-subscription-and-usage-for-your-enterprise-account)." For more information about managing your {% data variables.product.prodname_ghe_server %} billing settings, see "[Managing billing for your enterprise](/admin/overview/managing-billing-for-your-enterprise)."
+Enterprise accounts are currently available to {% data variables.product.prodname_ghe_cloud %} and {% data variables.product.prodname_ghe_server %} customers paying by invoice. Billing for all of the organizations and {% data variables.product.prodname_ghe_server %} instances connected to your enterprise account is aggregated into a single bill.
diff --git a/translations/ru-RU/data/reusables/enterprise_installation/hardware-considerations-all-platforms.md b/translations/ru-RU/data/reusables/enterprise_installation/hardware-considerations-all-platforms.md
index b3e2e9022c5a..1ef7257106f6 100644
--- a/translations/ru-RU/data/reusables/enterprise_installation/hardware-considerations-all-platforms.md
+++ b/translations/ru-RU/data/reusables/enterprise_installation/hardware-considerations-all-platforms.md
@@ -1,4 +1,5 @@
- [Minimum requirements](#minimum-requirements)
+- [Beta features in {% data variables.product.prodname_ghe_server %} 2.22](#beta-features-in-github-enterprise-server-222)
- [Storage](#storage)
- [CPU and memory](#cpu-and-memory)
@@ -6,23 +7,18 @@
We recommend different hardware configurations depending on the number of user licenses for {% data variables.product.product_location %}. If you provision more resources than the minimum requirements, your instance will perform and scale better.
-{% data reusables.enterprise_installation.hardware-rec-table %} For more information about adjusting resources for an existing instance, see "[Increasing storage capacity](/enterprise/admin/installation/increasing-storage-capacity)" and "[Increasing CPU or memory resources](/enterprise/admin/installation/increasing-cpu-or-memory-resources)."
-
-{% if currentVersion == "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
-
-If you enable the beta for {% data variables.product.prodname_actions %} on your instance, we recommend planning for additional capacity.
+{% data reusables.enterprise_installation.hardware-rec-table %}{% if currentVersion == "enterprise-server@2.22" or currentVersion == "github-ae@latest" %} If you enable the beta for {% data variables.product.prodname_actions %}, review the following requirements and recommendations.
- You must configure at least one runner for {% data variables.product.prodname_actions %} workflows. For more information, see "[About self-hosted runners](/actions/hosting-your-own-runners/about-self-hosted-runners)."
- You must configure external blob storage. For more information, see "[Enabling {% data variables.product.prodname_actions %} and configuring storage](/enterprise/admin/github-actions/enabling-github-actions-and-configuring-storage)."
-
-The additional CPU and memory resources you need to provision for your instance depend on the number of workflows your users run concurrently, and the overall levels of activity for users, automations, and integrations.
-
-| Maximum jobs per minute | vCPUs | Memory |
-|:----------------------- | -----:| -------:|
-| Light testing | 4 | 30.5 GB |
-| 25 | 8 | 61 GB |
-| 35 | 16 | 122 GB |
-| 100 | 32 | 244 GB |
+- You may need to configure additional CPU and memory resources. The additional resources you need to provision for {% data variables.product.prodname_actions %} depend on the number of workflows your users run concurrently, and the overall levels of activity for users, automations, and integrations.
+
+ | Maximum jobs per minute | Additional vCPUs | Additional memory |
+ |:----------------------- | ----------------:| -----------------:|
+ | Light testing | 4 | 30.5 GB |
+ | 25 | 8 | 61 GB |
+ | 35 | 16 | 122 GB |
+ | 100 | 32 | 244 GB |
{% endif %}
diff --git a/translations/ru-RU/data/reusables/enterprise_installation/hardware-rec-table.md b/translations/ru-RU/data/reusables/enterprise_installation/hardware-rec-table.md
index 4de4ad712004..97997e7d4f3a 100644
--- a/translations/ru-RU/data/reusables/enterprise_installation/hardware-rec-table.md
+++ b/translations/ru-RU/data/reusables/enterprise_installation/hardware-rec-table.md
@@ -1,6 +1,12 @@
{% if currentVersion == "enterprise-server@2.22" %}
-Minimum requirements for an instance with beta features enabled are **bold** in the following table. For more information, see "[Beta features in {% data variables.product.prodname_ghe_server %} 2.22](#beta-features-in-github-enterprise-server-222)." |{% endif %}
+{% note %}
+
+**Note**: If you enable beta features for {% data variables.product.prodname_ghe_server %} 2.22, your instance requires additional hardware resources. Minimum requirements for an instance with beta features enabled are **bold** in the following table. For more information about the features in beta, see "[Beta features in {% data variables.product.prodname_ghe_server %} 2.22](#beta-features-in-github-enterprise-server-222)."
+
+{% endnote %}
+|
+{% endif %}
| User licenses | vCPUs | Memory | Attached storage | Root storage |
|:------------------------------ | --------------------------------------------------------------------------------------------------------------------------------------:| ----------------------------------------------------------------------------------------------------------------------------------------------:| ----------------------------------------------------------------------------------------------------------------------------------------------:| ------------:|
| Trial, demo, or 10 light users | 2{% if currentVersion == "enterprise-server@2.22" %}
or [**4**](#beta-features-in-github-enterprise-server-222){% endif %} | 16 GB{% if currentVersion == "enterprise-server@2.22" %}
or [**32 GB**](#beta-features-in-github-enterprise-server-222){% endif %} | 100 GB{% if currentVersion == "enterprise-server@2.22" %}
or [**150 GB**](#beta-features-in-github-enterprise-server-222){% endif %} | 200 GB |
@@ -9,8 +15,14 @@ Minimum requirements for an instance with beta features enabled are **bold** in
| 5,000 to 8000 | 12{% if currentVersion == "enterprise-server@2.22" %}
or [**16**](#beta-features-in-github-enterprise-server-222){% endif %} | 96 GB | 750 GB | 200 GB |
| 8,000 to 10,000+ | 16{% if currentVersion == "enterprise-server@2.22" %}
or [**20**](#beta-features-in-github-enterprise-server-222){% endif %} | 128 GB{% if currentVersion == "enterprise-server@2.22" %}
or [**160 GB**](#beta-features-in-github-enterprise-server-222){% endif %} | 1000 GB | 200 GB |
+For more information about adjusting resources for an existing instance, see "[Increasing storage capacity](/enterprise/admin/installation/increasing-storage-capacity)" and "[Increasing CPU or memory resources](/enterprise/admin/installation/increasing-cpu-or-memory-resources)."
+
{% if currentVersion == "enterprise-server@2.22" %}
#### Beta features in {% data variables.product.prodname_ghe_server %} 2.22
-If you enable beta features in {% data variables.product.prodname_ghe_server %} 2.22, your instance requires additional hardware resources. For more information about the beta features, see the [release notes for the 2.22 series](https://enterprise.github.com/releases/series/2.22) on the {% data variables.product.prodname_enterprise %} website.{% endif %}
+You can sign up for beta features available in {% data variables.product.prodname_ghe_server %} 2.22 such as {% data variables.product.prodname_actions %}, {% data variables.product.prodname_registry %}, and {% data variables.product.prodname_code_scanning %}. For more information, see the [release notes for the 2.22 series](https://enterprise.github.com/releases/series/2.22#release-2.22.0) on the {% data variables.product.prodname_enterprise %} website.
+
+If you enable beta features for {% data variables.product.prodname_ghe_server %} 2.22, your instance requires additional hardware resources. For more information, see "[Minimum requirements](#minimum-requirements)".
+
+{% endif %}
diff --git a/translations/ru-RU/data/reusables/enterprise_installation/image-urls-viewable-warning.md b/translations/ru-RU/data/reusables/enterprise_installation/image-urls-viewable-warning.md
index 19b842266e07..2a26ad6d64f7 100644
--- a/translations/ru-RU/data/reusables/enterprise_installation/image-urls-viewable-warning.md
+++ b/translations/ru-RU/data/reusables/enterprise_installation/image-urls-viewable-warning.md
@@ -1,5 +1,5 @@
{% warning %}
-**Warning:** If you add an image attachment to a pull request or issue comment, anyone can view the anonymized image URL without authentication, even if the pull request is in a private repository, or if private mode is enabled. To keep sensitive images private, serve them from a private network or server that requires authentication.
+**Warning:** If you add an image attachment to a pull request or issue comment, anyone can view the anonymized image URL without authentication{% if enterpriseServerVersions contains currentVersion %}, even if the pull request is in a private repository, or if private mode is enabled. To prevent unauthorized access to the images, ensure that you restrict network access to the systems that serve the images, including {% data variables.product.product_location %}{% endif %}.{% if currentVersion == "github-ae@latest" %} To prevent unauthorized access to image URLs on {% data variables.product.product_name %}, consider restricting network traffic to your enterprise. For more information, see "[Restricting network traffic to your enterprise](/admin/configuration/restricting-network-traffic-to-your-enterprise)."{% endif %}
{% endwarning %}
diff --git a/translations/ru-RU/data/reusables/gated-features/pages.md b/translations/ru-RU/data/reusables/gated-features/pages.md
index e24925cc9877..31caf392d2d3 100644
--- a/translations/ru-RU/data/reusables/gated-features/pages.md
+++ b/translations/ru-RU/data/reusables/gated-features/pages.md
@@ -1 +1 @@
-{% data variables.product.prodname_pages %} is available in public repositories with {% data variables.product.prodname_free_user %} and {% data variables.product.prodname_free_team %} for organizations, and in public and private repositories with {% data variables.product.prodname_pro %}, {% data variables.product.prodname_team %}, {% data variables.product.prodname_ghe_cloud %},{% if currentVersion == "github-ae@latest" %} {% data variables.product.prodname_ghe_managed %},{% endif %} and {% data variables.product.prodname_ghe_server %}. {% if currentVersion == "free-pro-team@latest" %}{% data reusables.gated-features.more-info %}{% endif %}
+{% if currentVersion == "github-ae@latest" %}{% data variables.product.prodname_pages %} is available in internal and private repositories with {% data variables.product.prodname_ghe_managed %}. {% endif %}{% data variables.product.prodname_pages %} is available in public repositories with {% data variables.product.prodname_free_user %} and {% data variables.product.prodname_free_team %} for organizations, and in public and private repositories with {% data variables.product.prodname_pro %}, {% data variables.product.prodname_team %}, {% data variables.product.prodname_ghe_cloud %}, and {% data variables.product.prodname_ghe_server %}. {% if currentVersion == "free-pro-team@latest" %}{% data reusables.gated-features.more-info %}{% endif %}
diff --git a/translations/ru-RU/data/reusables/gated-features/wikis.md b/translations/ru-RU/data/reusables/gated-features/wikis.md
index 61f560896e02..d7611dc3638c 100644
--- a/translations/ru-RU/data/reusables/gated-features/wikis.md
+++ b/translations/ru-RU/data/reusables/gated-features/wikis.md
@@ -1 +1 @@
-Wikis are available in public repositories with {% data variables.product.prodname_free_user %} and {% data variables.product.prodname_free_team %} for organizations, and in public and private repositories with {% data variables.product.prodname_pro %}, {% data variables.product.prodname_team %}, {% data variables.product.prodname_ghe_cloud %},{% if currentVersion == "github-ae@latest" %} {% data variables.product.prodname_ghe_managed %},{% endif %} and {% data variables.product.prodname_ghe_server %}. {% if currentVersion == "free-pro-team@latest" %}{% data reusables.gated-features.more-info %}{% endif %}
+{% if currentVersion == "github-ae@latest" %}Wikis are available in internal and private repositories with {% data variables.product.prodname_ghe_managed %}.{% endif %} Wikis are available in public repositories with {% data variables.product.prodname_free_user %} and {% data variables.product.prodname_free_team %} for organizations, and in public and private repositories with {% data variables.product.prodname_pro %}, {% data variables.product.prodname_team %}, {% data variables.product.prodname_ghe_cloud %} and {% data variables.product.prodname_ghe_server %}. {% if currentVersion == "free-pro-team@latest" %}{% data reusables.gated-features.more-info %}{% endif %}
diff --git a/translations/ru-RU/data/reusables/github-actions/self-hosted-runner-configure-runner-group-access.md b/translations/ru-RU/data/reusables/github-actions/self-hosted-runner-configure-runner-group-access.md
index 59d3343c244e..9ac93498fa1e 100644
--- a/translations/ru-RU/data/reusables/github-actions/self-hosted-runner-configure-runner-group-access.md
+++ b/translations/ru-RU/data/reusables/github-actions/self-hosted-runner-configure-runner-group-access.md
@@ -1,2 +1,12 @@
-1. In the **Self-hosted runners** section of the settings page, click {% octicon "kebab-horizontal" aria-label="The horizontal kebab icon" %} next to the runner group you'd like to configure, then click **Edit name and [organization|repository] access**. 
-1. Select a new policy from the dropdown list, or modify the runner group name.
+1. In the **Self-hosted runners** section of the settings page, click {% octicon "kebab-horizontal" aria-label="The horizontal kebab icon" %} next to the runner group you'd like to configure, then click **Edit name and [organization|repository] access**.
+ 
+1. Modify your policy options, or change the runner group name.
+
+ {% warning %}
+
+ **Warning**
+ {% indented_data_reference site.data.reusables.github-actions.self-hosted-runner-security spaces=3 %}
+ For more information, see "[About self-hosted runners](/actions/hosting-your-own-runners/about-self-hosted-runners#self-hosted-runner-security-with-public-repositories)."
+
+ {% endwarning %}
+
diff --git a/translations/ru-RU/data/reusables/notifications-v2/custom-notifications-beta.md b/translations/ru-RU/data/reusables/notifications-v2/custom-notifications-beta.md
new file mode 100644
index 000000000000..dbcee80cfe1d
--- /dev/null
+++ b/translations/ru-RU/data/reusables/notifications-v2/custom-notifications-beta.md
@@ -0,0 +1,9 @@
+{% if currentVersion == "free-pro-team@latest" %}
+
+{% note %}
+
+**Note:** Custom notifications are currently in beta and subject to change.
+
+{% endnote %}
+
+{% endif %}
diff --git a/translations/ru-RU/data/reusables/notifications/outbound_email_tip.md b/translations/ru-RU/data/reusables/notifications/outbound_email_tip.md
index 6e261ac61517..1cd91b0775f1 100644
--- a/translations/ru-RU/data/reusables/notifications/outbound_email_tip.md
+++ b/translations/ru-RU/data/reusables/notifications/outbound_email_tip.md
@@ -1,7 +1,9 @@
-{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
- {% tip %}
+{% if enterpriseServerVersions contains currentVersion %}
- You'll only receive email notifications if outbound email support is enabled on {% data variables.product.product_location %}. For more information, contact your site administrator.
+{% note %}
+
+**Note**: You'll only receive email notifications if outbound email support is enabled on {% data variables.product.product_location %}. For more information, contact your site administrator.
+
+{% endnote %}
- {% endtip %}
{% endif %}
diff --git a/translations/ru-RU/data/reusables/notifications/shared_state.md b/translations/ru-RU/data/reusables/notifications/shared_state.md
index f9a4d8b42757..d6b53f667575 100644
--- a/translations/ru-RU/data/reusables/notifications/shared_state.md
+++ b/translations/ru-RU/data/reusables/notifications/shared_state.md
@@ -1,5 +1,5 @@
{% tip %}
-**Tip:** If you receive both web and email notifications, you can automatically sync the read or unread status of the notification so that web notifications are automatically marked as read once you've read the corresponding email notification. To enable this sync, your email client must be able to view images from {% if currentVersion == "free-pro-team@latest" %}'`notifications@github.com`'{% else %}'the no-reply email address configured by your site administrator'{% endif %}.
+**Tip:** If you receive both web and email notifications, you can automatically sync the read or unread status of the notification so that web notifications are automatically marked as read once you've read the corresponding email notification. To enable this sync, your email client must be able to view images from {% if currentVersion == "free-pro-team@latest" %}`notifications@github.com`{% else %}the `no-reply` email address {% if currentVersion == "github-ae@latest" %}for your {% data variables.product.product_name %} hostname{% elsif enterpriseServerVersions contains currentVersion %}for {% data variables.product.product_location %}, which your site administrator configures{% endif %}{% endif %}.
{% endtip %}
diff --git a/translations/ru-RU/data/reusables/profile/profile-readme.md b/translations/ru-RU/data/reusables/profile/profile-readme.md
index a19a3d4a30d3..7350ed57bd30 100644
--- a/translations/ru-RU/data/reusables/profile/profile-readme.md
+++ b/translations/ru-RU/data/reusables/profile/profile-readme.md
@@ -1 +1 @@
-If you add a README file to the root of a public repository with the same name as your username, that README will automatically appear on your profile page. You can edit your profile README with GitHub Flavored Markdown to create a personalized section on your profile.
+If you add a README file to the root of a public repository with the same name as your username, that README will automatically appear on your profile page. You can edit your profile README with {% data variables.product.company_short %} Flavored Markdown to create a personalized section on your profile. For more information, see "[Managing your profile README](/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme)."
diff --git a/translations/ru-RU/data/reusables/repositories/administrators-can-disable-issues.md b/translations/ru-RU/data/reusables/repositories/administrators-can-disable-issues.md
new file mode 100644
index 000000000000..19c435397a28
--- /dev/null
+++ b/translations/ru-RU/data/reusables/repositories/administrators-can-disable-issues.md
@@ -0,0 +1 @@
+Repository administrators can disable issues for a repository. For more information, see "[Disabling issues](/github/managing-your-work-on-github/disabling-issues)."
diff --git a/translations/ru-RU/data/reusables/repositories/desktop-fork.md b/translations/ru-RU/data/reusables/repositories/desktop-fork.md
index cf7bb2d54690..c7d52b1a88b1 100644
--- a/translations/ru-RU/data/reusables/repositories/desktop-fork.md
+++ b/translations/ru-RU/data/reusables/repositories/desktop-fork.md
@@ -1 +1 @@
-You can use {% data variables.product.prodname_desktop %} to fork a repository. For more information, see “[Cloning and forking repositories from {% data variables.product.prodname_desktop %}](/desktop/contributing-to-projects/cloning-and-forking-repositories-from-github-desktop)."
+You can use {% data variables.product.prodname_desktop %} to fork a repository. For more information, see "[Cloning and forking repositories from {% data variables.product.prodname_desktop %}](/desktop/contributing-to-projects/cloning-and-forking-repositories-from-github-desktop)."
diff --git a/translations/ru-RU/data/reusables/repositories/you-can-fork.md b/translations/ru-RU/data/reusables/repositories/you-can-fork.md
index 2d290ce6cde4..e72953648ddb 100644
--- a/translations/ru-RU/data/reusables/repositories/you-can-fork.md
+++ b/translations/ru-RU/data/reusables/repositories/you-can-fork.md
@@ -1,3 +1,7 @@
-You can fork any public repository to your user account or any organization where you have repository creation permissions. For more information, see "[Permission levels for an organization](/articles/permission-levels-for-an-organization)."
+{% if currentVersion == "github-ae@latest" %}If the policies for your enterprise permit forking internal and private repositories, you{% else %}You{% endif %} can fork a repository to your user account or any organization where you have repository creation permissions. For more information, see "[Permission levels for an organization](/articles/permission-levels-for-an-organization)."
-You can fork any private repository you can access to your user account and any organization on {% data variables.product.prodname_team %} or {% data variables.product.prodname_enterprise %} where you have repository creation permissions. You cannot fork a private repository to an organization using {% data variables.product.prodname_free_team %}.{% if currentVersion == "free-pro-team@latest" %} For more information, see "[GitHub's products](/articles/githubs-products)."{% endif %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+If you have access to a private repository and the owner permits forking, you can fork the repository to your user account or any organization on {% if currentVersion == "free-pro-team@latest"%}{% data variables.product.prodname_team %}{% else %}{% data variables.product.product_location %}{% endif %} where you have repository creation permissions. {% if currentVersion == "free-pro-team@latest" %}You cannot fork a private repository to an organization using {% data variables.product.prodname_free_team %}. For more information, see "[GitHub's products](/articles/githubs-products)."{% endif %}
+
+{% endif %}
\ No newline at end of file
diff --git a/translations/ru-RU/data/reusables/search/required_login.md b/translations/ru-RU/data/reusables/search/required_login.md
index 813810508ced..6d8598a94a38 100644
--- a/translations/ru-RU/data/reusables/search/required_login.md
+++ b/translations/ru-RU/data/reusables/search/required_login.md
@@ -1 +1 @@
-You must be signed in to search for code across all public repositories.
+You must be signed into a user account on {% data variables.product.product_name %} to search for code across all public repositories.
diff --git a/translations/ru-RU/data/reusables/search/syntax_tips.md b/translations/ru-RU/data/reusables/search/syntax_tips.md
index 9470b7f01a9d..363da832722a 100644
--- a/translations/ru-RU/data/reusables/search/syntax_tips.md
+++ b/translations/ru-RU/data/reusables/search/syntax_tips.md
@@ -1,7 +1,7 @@
{% tip %}
**Tips:**{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
- - This article contains example searches on the {% data variables.product.prodname_dotcom %}.com website, but you can use the same search filters on {% data variables.product.product_location %}.{% endif %}
+ - This article contains links to example searches on the {% data variables.product.prodname_dotcom_the_website %} website, but you can use the same search filters with {% data variables.product.product_name %}. In the linked example searches, replace `github.com` with the hostname for {% data variables.product.product_location %}.{% endif %}
- For a list of search syntaxes that you can add to any search qualifier to further improve your results, see "[Understanding the search syntax](/articles/understanding-the-search-syntax)".
- Use quotations around multi-word search terms. For example, if you want to search for issues with the label "In progress," you'd search for `label:"in progress"`. Search is not case sensitive.
diff --git a/translations/ru-RU/data/reusables/search/you-can-search-globally.md b/translations/ru-RU/data/reusables/search/you-can-search-globally.md
new file mode 100644
index 000000000000..dd690bc80fe1
--- /dev/null
+++ b/translations/ru-RU/data/reusables/search/you-can-search-globally.md
@@ -0,0 +1 @@
+You can search globally across all of {% data variables.product.product_name %}, or scope your search to a particular repository or organization.
diff --git a/translations/ru-RU/data/reusables/ssh/add-ssh-key-to-ssh-agent-commandline.md b/translations/ru-RU/data/reusables/ssh/add-ssh-key-to-ssh-agent-commandline.md
index af4760bd1725..415f86736de3 100644
--- a/translations/ru-RU/data/reusables/ssh/add-ssh-key-to-ssh-agent-commandline.md
+++ b/translations/ru-RU/data/reusables/ssh/add-ssh-key-to-ssh-agent-commandline.md
@@ -1,3 +1,3 @@
```shell
-$ ssh-add ~/.ssh/id_rsa
+$ ssh-add ~/.ssh/id_ed25519
```
diff --git a/translations/ru-RU/data/reusables/ssh/add-ssh-key-to-ssh-agent.md b/translations/ru-RU/data/reusables/ssh/add-ssh-key-to-ssh-agent.md
index 6a2f98182365..fb1ca5ec3428 100644
--- a/translations/ru-RU/data/reusables/ssh/add-ssh-key-to-ssh-agent.md
+++ b/translations/ru-RU/data/reusables/ssh/add-ssh-key-to-ssh-agent.md
@@ -1 +1 @@
-If you created your key with a different name, or if you are adding an existing key that has a different name, replace *id_rsa* in the command with the name of your private key file.
+If you created your key with a different name, or if you are adding an existing key that has a different name, replace *id_ed25519* in the command with the name of your private key file.
diff --git a/translations/ru-RU/data/variables/action_code_examples.yml b/translations/ru-RU/data/variables/action_code_examples.yml
index 9cc8394aff49..37602b477502 100644
--- a/translations/ru-RU/data/variables/action_code_examples.yml
+++ b/translations/ru-RU/data/variables/action_code_examples.yml
@@ -6,7 +6,7 @@
href: actions/starter-workflows
tags:
- official
- - workflows
+ - рабочий процесс
-
title: Example services
description: Example workflows using service containers
@@ -64,7 +64,7 @@
- publishing
-
title: GitHub Project Automation+
- description: Automate GitHub Project cards with any webhook event.
+ description: Automate GitHub Project cards with any webhook event
languages: JavaScript
href: alex-page/github-project-automation-plus
tags:
@@ -131,8 +131,7 @@
- publishing
-
title: Label your Pull Requests auto-magically (using committed files)
- description: >-
- Github action to label your pull requests auto-magically (using committed files)
+ description: Github action to label your pull requests auto-magically (using committed files)
languages: 'TypeScript, Dockerfile, JavaScript'
href: Decathlon/pull-request-labeler-action
tags:
@@ -147,3 +146,191 @@
tags:
- запрос на включение внесенных изменений
- labels
+-
+ title: Get a list of file changes with a PR/Push
+ description: This action gives you will get outputs of the files that have changed in your repository
+ languages: 'TypeScript, Shell, JavaScript'
+ href: trilom/file-changes-action
+ tags:
+ - рабочий процесс
+ - репозиторий
+-
+ title: Private actions in any workflow
+ description: Allows private GitHub Actions to be easily reused
+ languages: 'TypeScript, JavaScript, Shell'
+ href: InVisionApp/private-action-loader
+ tags:
+ - рабочий процесс
+ - tools
+-
+ title: Label your issues using the issue's contents
+ description: A GitHub Action to automatically tag issues with labels and assignees
+ languages: 'JavaScript, TypeScript'
+ href: damccorm/tag-ur-it
+ tags:
+ - рабочий процесс
+ - tools
+ - labels
+ - issues
+-
+ title: Rollback a GitHub Release
+ description: A GitHub Action to rollback or delete a release
+ languages: 'JavaScript'
+ href: author/action-rollback
+ tags:
+ - рабочий процесс
+ - релизы
+-
+ title: Lock closed issues and Pull Requests
+ description: GitHub Action that locks closed issues and pull requests after a period of inactivity
+ languages: 'JavaScript'
+ href: dessant/lock-threads
+ tags:
+ - issues
+ - pull requests
+ - рабочий процесс
+-
+ title: Get Commit Difference Count Between Two Branches
+ description: This GitHub Action compares two branches and gives you the commit count between them
+ languages: 'JavaScript, Shell'
+ href: jessicalostinspace/commit-difference-action
+ tags:
+ - коммит
+ - разница
+ - рабочий процесс
+-
+ title: Generate Release Notes Based on Git References
+ description: GitHub Action to generate changelogs, and release notes
+ languages: 'JavaScript, Shell'
+ href: metcalfc/changelog-generator
+ tags:
+ - cicd
+ - release-notes
+ - рабочий процесс
+ - changelog
+-
+ title: Enforce Policies on GitHub Repositories and Commits
+ description: Policy enforcement for your pipelines
+ languages: 'Go, Makefile, Dockerfile, Shell'
+ href: talos-systems/conform
+ tags:
+ - докер
+ - build-automation
+ - рабочий процесс
+-
+ title: Auto Label Issue Based
+ description: Automatically label an issue based on the issue description
+ languages: 'TypeScript, JavaScript, Dockerfile'
+ href: Renato66/auto-label
+ tags:
+ - labels
+ - рабочий процесс
+ - automation
+-
+ title: Update Configured GitHub Actions to the Latest Versions
+ description: CLI tool to check whehter all your actions are up-to-date or not
+ languages: 'C#, Inno Setup, PowerSHell, Shell'
+ href: fabasoad/ghacu
+ tags:
+ - versions - Список с версиями и их зависимостями
+ - cli
+ - рабочий процесс
+-
+ title: Create Issue Branch
+ description: GitHub Action that automates the creation of issue branches
+ languages: 'JavaScript, Shell'
+ href: robvanderleek/create-issue-branch
+ tags:
+ - probot
+ - issues
+ - labels
+-
+ title: Remove old artifacts
+ description: Customize artifact cleanup
+ languages: 'JavaScript, Shell'
+ href: c-hive/gha-remove-artifacts
+ tags:
+ - artifacts
+ - рабочий процесс
+-
+ title: Sync Defined Files/Binaries to Wiki or External Repositories
+ description: GitHub Action to automatically sync changes to external repositories, like the wiki, for example
+ languages: 'Shell, Dockerfile'
+ href: kai-tub/external-repo-sync-action
+ tags:
+ - wiki
+ - sync
+ - рабочий процесс
+-
+ title: Create/Update/Delete a GitHub Wiki page based on any file
+ description: Updates your GitHub wiki by using rsync, allowing for exclusion of files and directories and actual deletion of files
+ languages: 'Shell, Dockerfile'
+ href: Andrew-Chen-Wang/github-wiki-action
+ tags:
+ - wiki
+ - докер
+ - рабочий процесс
+-
+ title: Prow GitHub Actions
+ description: Automation of policy enforcement, chat-ops, and automatic PR merging
+ languages: 'TypeScript, JavaScript'
+ href: jpmcb/prow-github-actions
+ tags:
+ - chat-ops
+ - prow
+ - рабочий процесс
+-
+ title: Check GitHub Status in your Workflow
+ description: Check GitHub Status in your workflow
+ languages: 'TypeScript, JavaScript'
+ href: crazy-max/ghaction-github-status
+ tags:
+ - состояние
+ - monitoring
+ - рабочий процесс
+-
+ title: Manage labels on GitHub as code
+ description: GitHub Action to manage labels (create/rename/update/delete)
+ languages: 'TypeScript, JavaScript'
+ href: crazy-max/ghaction-github-labeler
+ tags:
+ - labels
+ - рабочий процесс
+ - automation
+-
+ title: Distribute funding in free and open source projects
+ description: Continuous Distribution of funding to project contributors and dependencies
+ languages: 'Python, Docerfile, Shell, Ruby'
+ href: protontypes/libreselery
+ tags:
+ - sponsors
+ - funding
+ - payment
+-
+ title: Herald rules for GitHub
+ description: Add reviewers, subscribers, labels and assignees to your PR
+ languages: 'TypeScript, JavaScript'
+ href: gagoar/use-herald-action
+ tags:
+ - reviewers
+ - labels
+ - assignees
+ - запрос на включение внесенных изменений
+-
+ title: Codeowner validator
+ description: Ensures the correctness of your GitHub CODEOWNERS file, supports public and private GitHub repositories and also GitHub Enterprise installations
+ languages: 'Go, Shell, Makefile, Docerfile'
+ href: mszostok/codeowners-validator
+ tags:
+ - codeowners
+ - validate
+ - рабочий процесс
+-
+ title: Copybara Action
+ description: Move and transform code between repositories (ideal to maintain several repos from one monorepo)
+ languages: 'TypeScript, JavaScript, Shell'
+ href: olivr/copybara-action
+ tags:
+ - monorepo
+ - copybara
+ - рабочий процесс
diff --git a/translations/zh-CN/content/actions/hosting-your-own-runners/managing-access-to-self-hosted-runners-using-groups.md b/translations/zh-CN/content/actions/hosting-your-own-runners/managing-access-to-self-hosted-runners-using-groups.md
index b11f50d8ff21..d1641858552a 100644
--- a/translations/zh-CN/content/actions/hosting-your-own-runners/managing-access-to-self-hosted-runners-using-groups.md
+++ b/translations/zh-CN/content/actions/hosting-your-own-runners/managing-access-to-self-hosted-runners-using-groups.md
@@ -1,6 +1,6 @@
---
-title: 使用组管理自托管运行器的访问权限
-intro: 您可以使用策略来限制对已添加到组织或企业的自托管运行器的访问。
+title: Managing access to self-hosted runners using groups
+intro: You can use policies to limit access to self-hosted runners that have been added to an organization or enterprise.
redirect_from:
- /actions/hosting-your-own-runners/managing-access-to-self-hosted-runners
versions:
@@ -11,81 +11,102 @@ versions:
{% data reusables.actions.enterprise-beta %}
{% data reusables.actions.enterprise-github-hosted-runners %}
-### 关于自托管运行器组
+### About self-hosted runner groups
{% if currentVersion == "free-pro-team@latest" %}
{% note %}
-**注:**所有组织都有一个默认的自托管运行器组。 创建和管理其他自托管运行器组仅适用于企业帐户以及企业帐户拥有的组织。
+**Note:** All organizations have a single default self-hosted runner group. Creating and managing additional self-hosted runner groups is only available to enterprise accounts, and for organizations owned by an enterprise account.
{% endnote %}
{% endif %}
-自托管运行器组用于控制对组织和企业级自托管运行器的访问。 企业管理员可以配置访问策略,用以控制企业中的哪些组织可以访问运行器组。 组织管理员可以配置访问策略,用以控制组织中的哪些组织可以访问运行器组。
+Self-hosted runner groups are used to control access to self-hosted runners at the organization and enterprise level. Enterprise admins can configure access policies that control which organizations in an enterprise have access to the runner group. Organization admins can configure access policies that control which repositories in an organization have access to the runner group.
-当企业管理员授予组织对运行器组的访问权限时,组织管理员可以看到组织的自托管运行器设置中列出的运行器组。 然后,组织管理员可以为企业运行器组分配其他细致的仓库访问策略。
+When an enterprise admin grants an organization access to a runner group, organization admins can see the runner group listed in the organization's self-hosted runner settings. The organizations admins can then assign additional granular repository access policies to the enterprise runner group.
-新运行器在创建时,将自动分配给默认组。 运行器每次只能在一个组中。 您可以将运行器从默认组移到另一组。 更多信息请参阅“[将自托管运行器移动到组](#moving-a-self-hosted-runner-to-a-group)”。
+When new runners are created, they are automatically assigned to the default group. Runners can only be in one group at a time. You can move runners from the default group to another group. For more information, see "[Moving a self-hosted runner to a group](#moving-a-self-hosted-runner-to-a-group)."
-### 为组织创建自托管的运行器组
+### Creating a self-hosted runner group for an organization
-所有组织都有一个默认的自托管运行器组。 企业帐户中的组织可以创建其他自托管组。 组织管理员可以允许单个仓库访问运行器组。
+All organizations have a single default self-hosted runner group. Organizations within an enterprise account can create additional self-hosted groups. Organization admins can allow individual repositories access to a runner group.
-自托管运行器在创建时会自动分配给默认组,并且每次只能成为一个组的成员。 您可以将运行器从默认组移到您创建的任何组。
+Self-hosted runners are automatically assigned to the default group when created, and can only be members of one group at a time. You can move a runner from the default group to any group you create.
-创建组时,必须选择用于定义哪些仓库有权访问运行器组的策略。 您可以配置一个运行器组可供一组特定的仓库、所有私有仓库或组织中所有仓库访问。
+When creating a group, you must choose a policy that defines which repositories have access to the runner group.
{% data reusables.organizations.navigate-to-org %}
{% data reusables.organizations.org_settings %}
{% data reusables.organizations.settings-sidebar-actions %}
-1. 在 **Self-hosted runners(自托管运行器)**部分,单击 **Add new(新增)**,然后单击 **New group(新组)**。
+1. In the **Self-hosted runners** section, click **Add new**, and then **New group**.
- 
-1. 输入运行器组的名称,然后从“**Repository access(仓库访问)**”下拉列表中选择访问策略。
+ 
+1. Enter a name for your runner group, and assign a policy for repository access.
- 
-1. 单击 **Save group(保存组)**创建组并应用策略。
+ {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %} You can configure a runner group to be accessible to a specific list of repositories, or to all repositories in the organization. By default, public repositories can't access runners in a runner group, but you can use the **Allow public repositories** option to override this.{% else if currentVersion == "enterprise-server@2.22"%}You can configure a runner group to be accessible to a specific list of repositories, all private repositories, or all repositories in the organization.{% endif %}
-### 为企业创建自托管运行器组
+ {% warning %}
-企业可以将其自托管的运行器添加到组以进行访问管理。 企业可以创建供企业帐户中特定组织访问的自托管运行器组。 然后,组织管理员可以为企业运行器组分配其他细致的仓库访问策略。
+ **Warnung**
+ {% indented_data_reference site.data.reusables.github-actions.self-hosted-runner-security spaces=3 %}
+ Weitere Informationen findest Du unter „[Informationen zu selbst-gehosteten Runnern](/actions/hosting-your-own-runners/about-self-hosted-runners#self-hosted-runner-security-with-public-repositories)“.
-自托管运行器在创建时会自动分配给默认组,并且每次只能成为一个组的成员。 您可以在注册过程中将运行器分配给特定组,也可以稍后将运行器从默认组移到自定义组。
+ {% endwarning %}
-创建组时,必须选择一个策略以向企业中所有组织或所选特定组织授予访问权限。
+ 
+1. Click **Save group** to create the group and apply the policy.
+
+### Creating a self-hosted runner group for an enterprise
+
+Enterprises can add their self-hosted runners to groups for access management. Enterprises can create groups of self-hosted runners that are accessible to specific organizations in the enterprise account. Organization admins can then assign additional granular repository access policies to the enterprise runner groups.
+
+Self-hosted runners are automatically assigned to the default group when created, and can only be members of one group at a time. You can assign the runner to a specific group during the registration process, or you can later move the runner from the default group to a custom group.
+
+When creating a group, you must choose a policy that defines which organizations have access to the runner group.
{% data reusables.enterprise-accounts.access-enterprise %}
{% data reusables.enterprise-accounts.policies-tab %}
{% data reusables.enterprise-accounts.actions-tab %}
-1. 单击 **Self-hosted runners(自托管运行器)**选项卡。
-1. 单击 **Add new(新增)**,然后单击 **New group(新组)**。
+1. Click the **Self-hosted runners** tab.
+1. Click **Add new**, and then **New group**.
+
+ 
+1. Enter a name for your runner group, and assign a policy for organization access.
- 
-1. 输入运行器组的名称,然后从“**Organization access(组织访问)**”下拉列表中选择访问策略。
+ {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %} You can configure a runner group to be accessible to a specific list of organizations, or all organizations in the enterprise. By default, public repositories can't access runners in a runner group, but you can use the **Allow public repositories** option to override this.{% else if currentVersion == "enterprise-server@2.22"%}You can configure a runner group to be accessible to all organizations in the enterprise or choose specific organizations.{% endif %}
- 
-1. 单击 **Save group(保存组)**创建组并应用策略。
+ {% warning %}
-### 更改自托管运行器组的访问策略
+ **Warnung**
+ {% indented_data_reference site.data.reusables.github-actions.self-hosted-runner-security spaces=3 %}
+ Weitere Informationen findest Du unter „[Informationen zu selbst-gehosteten Runnern](/actions/hosting-your-own-runners/about-self-hosted-runners#self-hosted-runner-security-with-public-repositories)“.
-您可以更新运行器组的访问策略,或重命名运行器组。
+ {% endwarning %}
+
+ 
+1. Click **Save group** to create the group and apply the policy.
+
+### Changing the access policy of a self-hosted runner group
+
+You can update the access policy of a runner group, or rename a runner group.
{% data reusables.github-actions.self-hosted-runner-configure-runner-group-access %}
-### 将自托管的运行器移动到组
+### Moving a self-hosted runner to a group
+
+New self-hosted runners are automatically assigned to the default group, and can then be moved to another group.
-新的自托管运行器将自动分配给默认组,然后可以移到另一个组。
+1. In the **Self-hosted runners** section of the settings page, locate the current group of the runner you want to move group and expand the list of group members. 
+1. Select the checkbox next to the self-hosted runner, and then click **Move to group** to see the available destinations. 
+1. To move the runner, click on the destination group. 
-1. 在设置页面的 **Self-hosted runners(自托管运行器)**部分,找到要移动组的运行器的当前组,并展开组成员列表。 
-1. 选中自托管运行器旁边的复选框,然后单击 **Move to group(移动到组)**以查看可用的目的地。 
-1. 要移动运行器,请单击目标组。 
+### Removing a self-hosted runner group
-### 删除自托管运行器组
+Self-hosted runners are automatically returned to the default group when their group is removed.
-自托管运行器在其组被删除时将自动返回到默认组。
+1. In the **Self-hosted runners** section of the settings page, locate the group you want to delete, and click the {% octicon "kebab-horizontal" aria-label="The horizontal kebab icon" %} button. 
-1. 在设置页面的 **Self-hosted runners(自托管运行器)**部分,找到您想要删除的组,并单击 {% octicon "kebab-horizontal" aria-label="The horizontal kebab icon" %} 按钮。 
+1. To remove the group, click **Remove group**. 
-1. 要删除组,请单击 **Remove group(删除组)**。 
+1. Review the confirmation prompts, and click **Remove this runner group**.
-1. 查看确认提示,然后单击 **Remove this runner group(删除此运行器组)**。
diff --git a/translations/zh-CN/content/actions/reference/context-and-expression-syntax-for-github-actions.md b/translations/zh-CN/content/actions/reference/context-and-expression-syntax-for-github-actions.md
index cea7fe9d249c..d7251d04f159 100644
--- a/translations/zh-CN/content/actions/reference/context-and-expression-syntax-for-github-actions.md
+++ b/translations/zh-CN/content/actions/reference/context-and-expression-syntax-for-github-actions.md
@@ -123,7 +123,7 @@ env:
| 属性名称 | 类型 | 描述 |
| ----------------------------------------- | ----- | ------------------------------------------------------------------------------------------------------------------------------------------------------ |
-| `作业` | `对象` | 此上下文针对工作流程运行中的每项作业而改变。 您可以从作业中的任何步骤访问此上下文。 |
+| `job` | `对象` | 此上下文针对工作流程运行中的每项作业而改变。 您可以从作业中的任何步骤访问此上下文。 |
| `job.container` | `对象` | 作业的容器相关信息。 有关容器的更多信息,请参阅“[{% data variables.product.prodname_actions %} 的工作流程语法](/articles/workflow-syntax-for-github-actions#jobsjob_idcontainer)”。 |
| `job.container.id` | `字符串` | 容器的 id。 |
| `job.container.network` | `字符串` | 容器网络的 id。 运行程序创建作业中所有容器使用的网络。 |
diff --git a/translations/zh-CN/content/actions/reference/events-that-trigger-workflows.md b/translations/zh-CN/content/actions/reference/events-that-trigger-workflows.md
index 826301cad378..124fa1ab0d7f 100644
--- a/translations/zh-CN/content/actions/reference/events-that-trigger-workflows.md
+++ b/translations/zh-CN/content/actions/reference/events-that-trigger-workflows.md
@@ -572,6 +572,8 @@ on:
{% data reusables.developer-site.pull_request_forked_repos_link %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %}
+
#### `pull_request_target`
此事件类似于 `pull_request`,只不过它在拉取请求的基础仓库的上下文中运行,而不是在合并提交中运行。 这意味着您可以更安全地将您的密码提供给由拉取请求触发的工作流程,因为只有在基础仓库的提交中定义的工作流程才会运行。 例如,此事件允许您根据事件有效负载的内容创建工作流程来标识和评论拉取请求。
@@ -589,6 +591,8 @@ on: pull_request_target
types: [assigned, opened, synchronize, reopened]
```
+{% endif %}
+
#### `推送`
{% note %}
@@ -689,6 +693,8 @@ on:
types: [started]
```
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %}
+
#### `workflow_run`
{% data reusables.webhooks.workflow_run_desc %}
@@ -711,6 +717,8 @@ on:
- requested
```
+{% endif %}
+
### 使用个人访问令牌触发新工作流程
{% data reusables.github-actions.actions-do-not-trigger-workflows %} 更多信息请参阅“[使用 GITHUB_TOKEN 验证身份](/actions/configuring-and-managing-workflows/authenticating-with-the-github_token)”。
diff --git a/translations/zh-CN/content/actions/reference/workflow-syntax-for-github-actions.md b/translations/zh-CN/content/actions/reference/workflow-syntax-for-github-actions.md
index 46a9883e0750..2801fbbc44d8 100644
--- a/translations/zh-CN/content/actions/reference/workflow-syntax-for-github-actions.md
+++ b/translations/zh-CN/content/actions/reference/workflow-syntax-for-github-actions.md
@@ -876,6 +876,37 @@ strategy:
{% endnote %}
+##### Using environment variables in a matrix
+
+You can add custom environment variables for each test combination by using `include` with `env`. You can then refer to the custom environment variables in a later step.
+
+In this example, the matrix entries for `node-version` are each configured to use different values for the `site` and `datacenter` environment variables. The `Echo site details` step then uses {% raw %}`env: ${{ matrix.env }}`{% endraw %} to refer to the custom variables:
+
+{% raw %}
+```yaml
+name: Node.js CI
+on: [push]
+jobs:
+ build:
+ runs-on: ubuntu-latest
+ strategy:
+ matrix:
+ include:
+ - node-version: 10.x
+ site: "prod"
+ datacenter: "site-a"
+ - node-version: 12.x
+ site: "dev"
+ datacenter: "site-b"
+ steps:
+ - name: Echo site details
+ env:
+ SITE: ${{ matrix.site }}
+ DATACENTER: ${{ matrix.datacenter }}
+ run: echo $SITE $DATACENTER
+```
+{% endraw %}
+
### **`jobs..strategy.fail-fast`**
设置为 `true` 时,如果任何 `matrix` 作业失败,{% data variables.product.prodname_dotcom %} 将取消所有进行中的作业。 默认值:`true`
diff --git a/translations/zh-CN/content/admin/configuration/command-line-utilities.md b/translations/zh-CN/content/admin/configuration/command-line-utilities.md
index 2a777ef1605c..0da28a89c1b4 100644
--- a/translations/zh-CN/content/admin/configuration/command-line-utilities.md
+++ b/translations/zh-CN/content/admin/configuration/command-line-utilities.md
@@ -84,7 +84,7 @@ $ ghe-config -l
允许您将用户列表从 API 速率限制中排除。 更多信息请参阅“[REST API 中的资源](/rest/overview/resources-in-the-rest-api#rate-limiting)”。
``` shell
-$ ghe-config app.github.rate_limiting_exempt_users "hubot github-actions"
+$ ghe-config app.github.rate-limiting-exempt-users "hubot github-actions"
# Exempts the users hubot and github-actions from rate limits
```
{% endif %}
diff --git a/translations/zh-CN/content/admin/enterprise-management/configuring-high-availability-replication-for-a-cluster.md b/translations/zh-CN/content/admin/enterprise-management/configuring-high-availability-replication-for-a-cluster.md
index 902f80f5f05c..b219fe0cb21c 100644
--- a/translations/zh-CN/content/admin/enterprise-management/configuring-high-availability-replication-for-a-cluster.md
+++ b/translations/zh-CN/content/admin/enterprise-management/configuring-high-availability-replication-for-a-cluster.md
@@ -57,32 +57,36 @@ versions:
mysql-master = HOSTNAME
redis-master = HOSTNAME
primary-datacenter = default
- ```
+ ```
- (可选)通过编辑 `primary-datacenter` 的值,将主数据中心的名称更改为更具描述性或更准确的值。
4. {% data reusables.enterprise_clustering.configuration-file-heading %} 在每个节点标题下,添加新的键值对,以将节点分配给数据中心。 使用与上述步骤 3 的 `primary-datacenter` 相同的值。 例如,如果要使用默认名称 (`default`),请将以下键值对添加到每个节点的部分。
- datacenter = default
+ ```
+ datacenter = default
+ ```
完成后,群集配置文件中每个节点的部分应如下所示。 {% data reusables.enterprise_clustering.key-value-pair-order-irrelevant %}
- ```shell
- [cluster "HOSTNAME"]
- datacenter = default
- hostname = HOSTNAME
- ipv4 = IP ADDRESS
+ ```shell
+ [cluster "HOSTNAME"]
+ datacenter = default
+ hostname = HOSTNAME
+ ipv4 = IP ADDRESS
+ ...
...
- ...
- ```
+ ```
- {% note %}
+ {% note %}
- **注**:如果在步骤 3 中更改了主数据中心的名称,请在每个节点的部分找到 `consul-datacenter` 键值对,然后将值更改为重命名的主数据中心。 例如,如果您将主数据中心命名为 `primary`,则对每个节点使用以下键值对。
+ **注**:如果在步骤 3 中更改了主数据中心的名称,请在每个节点的部分找到 `consul-datacenter` 键值对,然后将值更改为重命名的主数据中心。 例如,如果您将主数据中心命名为 `primary`,则对每个节点使用以下键值对。
- consul-datacenter = primary
+ ```
+ consul-datacenter = primary
+ ```
- {% endnote %}
+ {% endnote %}
{% data reusables.enterprise_clustering.apply-configuration %}
@@ -103,31 +107,37 @@ versions:
1. 对于群集中的每个节点,预配规范相同的匹配虚拟机,运行相同版本的 {% data variables.product.prodname_ghe_server %}。 记下每个新群集节点的 IPv4 地址和主机名。 更多信息请参阅“[先决条件](#prerequisites)”。
- {% note %}
+ {% note %}
- **注**:如果在故障转移后重新配置高可用性,可以改为使用主数据中心的旧节点。
+ **注**:如果在故障转移后重新配置高可用性,可以改为使用主数据中心的旧节点。
- {% endnote %}
+ {% endnote %}
{% data reusables.enterprise_clustering.ssh-to-a-node %}
3. 备份现有群集配置。
-
- cp /data/user/common/cluster.conf ~/$(date +%Y-%m-%d)-cluster.conf.backup
+
+ ```
+ cp /data/user/common/cluster.conf ~/$(date +%Y-%m-%d)-cluster.conf.backup
+ ```
4. 在临时位置创建现有群集配置文件的副本,如 _/home/admin/cluster-passive.conf_。 删除 IP 地址的唯一键值对 (`ipv*`)、UUID (`uuid`) 和 WireGuard 的公钥 (`wireguard-pubkey`)。
-
- grep -Ev "(?:|ipv|uuid|vpn|wireguard\-pubkey)" /data/user/common/cluster.conf > ~/cluster-passive.conf
+
+ ```
+ grep -Ev "(?:|ipv|uuid|vpn|wireguard\-pubkey)" /data/user/common/cluster.conf > ~/cluster-passive.conf
+ ```
5. 从上一步复制的临时群集配置文件中删除 `[cluster]` 部分。
-
- git config -f ~/cluster-passive.conf --remove-section cluster
+
+ ```
+ git config -f ~/cluster-passive.conf --remove-section cluster
+ ```
6. 确定在其中预配了被动节点的辅助数据中心的名称,然后使用新的数据中心名称更新临时群集配置文件。 将 `SECONDARY` 替换为您选择的名称。
```shell
- sed -i 's/datacenter = default/datacenter = SECONDARY/g' ~/cluster-passive.conf
- ```
+ sed -i 's/datacenter = default/datacenter = SECONDARY/g' ~/cluster-passive.conf
+ ```
7. 确定被动节点主机名的模式。
@@ -140,7 +150,7 @@ versions:
8. 在文本编辑器中打开步骤 3 中的临时群集配置文件。 例如,您可以使用 Vim。
```shell
- sudo vim ~/cluster-passive.conf
+ sudo vim ~/cluster-passive.conf
```
9. 在临时群集配置文件中的每个部分,更新节点的配置。 {% data reusables.enterprise_clustering.configuration-file-heading %}
@@ -150,37 +160,37 @@ versions:
- 新增键值对 `replica = enabled`。
```shell
- [cluster "NEW PASSIVE NODE HOSTNAME"]
+ [cluster "NEW PASSIVE NODE HOSTNAME"]
+ ...
+ hostname = NEW PASSIVE NODE HOSTNAME
+ ipv4 = NEW PASSIVE NODE IPV4 ADDRESS
+ replica = enabled
+ ...
...
- hostname = NEW PASSIVE NODE HOSTNAME
- ipv4 = NEW PASSIVE NODE IPV4 ADDRESS
- replica = enabled
- ...
- ...
```
10. 将步骤 4 中创建的临时群集配置文件的内容附加到活动的配置文件。
```shell
- cat ~/cluster-passive.conf >> /data/user/common/cluster.conf
- ```
+ cat ~/cluster-passive.conf >> /data/user/common/cluster.conf
+ ```
11. 在辅助数据中心中指定主 MySQL 和 Redis 节点。 将 `REPLICA MYSQL PRIMARY HOSTNAME` 和 `REPLICA REDIS PRIMARY HOSTNAME` 替换为您预配的被动节点的主机名,以匹配您现有的 MySQL 和 Redis 主节点。
```shell
- git config -f /data/user/common/cluster.conf cluster.mysql-master-replica REPLICA MYSQL PRIMARY HOSTNAME
- git config -f /data/user/common/cluster.conf cluster.redis-master-replica REPLICA REDIS PRIMARY HOSTNAME
- ```
+ git config -f /data/user/common/cluster.conf cluster.mysql-master-replica REPLICA MYSQL PRIMARY HOSTNAME
+ git config -f /data/user/common/cluster.conf cluster.redis-master-replica REPLICA REDIS PRIMARY HOSTNAME
+ ```
12. 启用 MySQL 在故障转移到被动副本节点时自动故障转移。
```shell
- git config -f /data/user/common/cluster.conf cluster.mysql-auto-failover true
+ git config -f /data/user/common/cluster.conf cluster.mysql-auto-failover true
```
- {% warning %}
+ {% warning %}
- **警告**:在继续操作之前查看群集配置文件。
+ **警告**:在继续操作之前查看群集配置文件。
- 在顶层 `[cluster]` 部分中,确保 `mysql-master-replica` 和 `redis-master-replica` 的值,是辅助数据中心中在故障转移后用作 MySQL 和 Redis 主节点的被动节点的正确主机名。
- 在名为 `[cluster "ACTIVE NODE HOSTNAME"]` 的主动节点的每个部分中,双击以下键值对。
@@ -194,9 +204,9 @@ versions:
- `replica` 应配置为 `enabled`。
- 利用机会删除已经不再使用的离线节点的部分。
- 要查看示例配置,请参阅“[示例配置](#example-configuration)”。
+ 要查看示例配置,请参阅“[示例配置](#example-configuration)”。
- {% endwarning %}
+ {% endwarning %}
13. 初始化新群集配置。 {% data reusables.enterprise.use-a-multiplexer %}
@@ -207,7 +217,7 @@ versions:
14. 初始化完成后,{% data variables.product.prodname_ghe_server %} 将显示以下消息。
```shell
- Finished cluster initialization
+ Finished cluster initialization
```
{% data reusables.enterprise_clustering.apply-configuration %}
@@ -293,20 +303,28 @@ versions:
您可以通过 {% data variables.product.prodname_ghe_server %} 系统管理 shell 使用命令行工具监控群集中任何节点的进度。 有关系统管理 shell 的更多信息,请参阅“[访问管理 shell (SSH)](/enterprise/admin/configuration/accessing-the-administrative-shell-ssh)。”
- 监控数据库的复制:
-
- /usr/local/share/enterprise/ghe-cluster-status-mysql
+
+ ```
+ /usr/local/share/enterprise/ghe-cluster-status-mysql
+ ```
- 监控仓库和 Gist 数据的复制:
-
- ghe-spokes status
+
+ ```
+ ghe-spokes status
+ ```
- 监控附件和 LFS 数据的复制:
-
- ghe-storage replication-status
+
+ ```
+ ghe-storage replication-status
+ ```
- 监控 Pages 数据的复制:
-
- ghe-dpages replication-status
+
+ ```
+ ghe-dpages replication-status
+ ```
您可以使用 `ghe-cluster-status` 来审查群集的总体健康状况。 更多信息请参阅“[命令行实用程序](/enterprise/admin/configuration/command-line-utilities#ghe-cluster-status)”。
diff --git a/translations/zh-CN/content/admin/enterprise-management/increasing-storage-capacity.md b/translations/zh-CN/content/admin/enterprise-management/increasing-storage-capacity.md
index 3a3ed54d79b5..46d74150b773 100644
--- a/translations/zh-CN/content/admin/enterprise-management/increasing-storage-capacity.md
+++ b/translations/zh-CN/content/admin/enterprise-management/increasing-storage-capacity.md
@@ -20,6 +20,8 @@ versions:
{% endnote %}
+#### 最低要求
+
{% data reusables.enterprise_installation.hardware-rec-table %}
### 增加数据分区大小
diff --git a/translations/zh-CN/content/admin/installation/installing-github-enterprise-server-on-google-cloud-platform.md b/translations/zh-CN/content/admin/installation/installing-github-enterprise-server-on-google-cloud-platform.md
index 9d5555cae038..ca42c161178b 100644
--- a/translations/zh-CN/content/admin/installation/installing-github-enterprise-server-on-google-cloud-platform.md
+++ b/translations/zh-CN/content/admin/installation/installing-github-enterprise-server-on-google-cloud-platform.md
@@ -26,14 +26,14 @@ versions:
以下 Google Compute Engine (GCE) 机器类型支持 {% data variables.product.prodname_ghe_server %}。 更多信息请参阅 [Google Cloud Platform 机器类型文章](https://cloud.google.com/compute/docs/machine-types)。
-| | 高内存 |
-| | ------------- |
-| | n1-highmem-4 |
-| | n1-highmem-8 |
-| | n1-highmem-16 |
-| | n1-highmem-32 |
-| | n1-highmem-64 |
-| | n1-highmem-96 |
+| 高内存 |
+| ------------- |
+| n1-highmem-4 |
+| n1-highmem-8 |
+| n1-highmem-16 |
+| n1-highmem-32 |
+| n1-highmem-64 |
+| n1-highmem-96 |
#### 建议的机器类型
@@ -54,7 +54,7 @@ versions:
1. 使用 [gcloud compute](https://cloud.google.com/compute/docs/gcloud-compute/) 命令行工具列出公共 {% data variables.product.prodname_ghe_server %} 映像:
```shell
$ gcloud compute images list --project github-enterprise-public --no-standard-images
- ```
+ ```
2. 记下 {% data variables.product.prodname_ghe_server %} 最新 GCE 映像的映像名称。
@@ -63,18 +63,18 @@ versions:
GCE 虚拟机作为具有防火墙的网络的成员创建。 对于与 {% data variables.product.prodname_ghe_server %} VM 关联的网络,您需要将防火墙配置为允许下表中列出的必需端口。 更多关于 Google Cloud Platform 上防火墙规则的信息,请参阅 Google 指南“[防火墙规则概述](https://cloud.google.com/vpc/docs/firewalls)”。
1. 使用 gcloud compute 命令行工具创建网络。 更多信息请参阅 Google 文档中的“[gcloud compute networks create](https://cloud.google.com/sdk/gcloud/reference/compute/networks/create)”。
- ```shell
- $ gcloud compute networks create NETWORK-NAME --subnet-mode auto
- ```
+ ```shell
+ $ gcloud compute networks create NETWORK-NAME --subnet-mode auto
+ ```
2. 为下表中的各个端口创建防火墙规则。 更多信息请参阅 Google 文档中的“[gcloud compute firewall-rules](https://cloud.google.com/sdk/gcloud/reference/compute/firewall-rules/)”。
- ```shell
- $ gcloud compute firewall-rules create RULE-NAME \
- --network NETWORK-NAME \
- --allow tcp:22,tcp:25,tcp:80,tcp:122,udp:161,tcp:443,udp:1194,tcp:8080,tcp:8443,tcp:9418,icmp
- ```
- 此表列出了必需端口以及各端口的用途。
+ ```shell
+ $ gcloud compute firewall-rules create RULE-NAME \
+ --network NETWORK-NAME \
+ --allow tcp:22,tcp:25,tcp:80,tcp:122,udp:161,tcp:443,udp:1194,tcp:8080,tcp:8443,tcp:9418,icmp
+ ```
+ 此表列出了必需端口以及各端口的用途。
- {% data reusables.enterprise_installation.necessary_ports %}
+ {% data reusables.enterprise_installation.necessary_ports %}
### 分配静态 IP 并将其分配给 VM
@@ -87,21 +87,21 @@ GCE 虚拟机作为具有防火墙的网络的成员创建。 对于与 {% data
要创建 {% data variables.product.prodname_ghe_server %} 实例,您需要使用 {% data variables.product.prodname_ghe_server %} 映像创建 GCE 实例并连接额外的存储卷来存储实例数据。 更多信息请参阅“[硬件考量因素](#hardware-considerations)”。
1. 使用 gcloud compute 命令行工具,创建数据磁盘,将其用作您的实例数据的附加存储卷,并根据用户许可数配置大小。 更多信息请参阅 Google 文档中的“[gcloud compute disks create](https://cloud.google.com/sdk/gcloud/reference/compute/disks/create)”。
- ```shell
- $ gcloud compute disks create DATA-DISK-NAME --size DATA-DISK-SIZE --type DATA-DISK-TYPE --zone ZONE
- ```
+ ```shell
+ $ gcloud compute disks create DATA-DISK-NAME --size DATA-DISK-SIZE --type DATA-DISK-TYPE --zone ZONE
+ ```
2. 然后,使用所选 {% data variables.product.prodname_ghe_server %} 映像的名称创建实例,并连接数据磁盘。 更多信息请参阅 Google 文档中的“[gcloud compute ](https://cloud.google.com/sdk/gcloud/reference/compute/instances/create)”。
- ```shell
- $ gcloud compute instances create INSTANCE-NAME \
- --machine-type n1-standard-8 \
- --image GITHUB-ENTERPRISE-IMAGE-NAME \
- --disk name=DATA-DISK-NAME \
- --metadata serial-port-enable=1 \
- --zone ZONE \
- --network NETWORK-NAME \
- --image-project github-enterprise-public
- ```
+ ```shell
+ $ gcloud compute instances create INSTANCE-NAME \
+ --machine-type n1-standard-8 \
+ --image GITHUB-ENTERPRISE-IMAGE-NAME \
+ --disk name=DATA-DISK-NAME \
+ --metadata serial-port-enable=1 \
+ --zone ZONE \
+ --network NETWORK-NAME \
+ --image-project github-enterprise-public
+ ```
### 配置实例
diff --git a/translations/zh-CN/content/admin/overview/about-enterprise-accounts.md b/translations/zh-CN/content/admin/overview/about-enterprise-accounts.md
index 778ee64ce917..7a6e917b80bb 100644
--- a/translations/zh-CN/content/admin/overview/about-enterprise-accounts.md
+++ b/translations/zh-CN/content/admin/overview/about-enterprise-accounts.md
@@ -1,26 +1,31 @@
---
title: 关于企业帐户
-intro: '使用 {% data variables.product.prodname_ghe_server %},可以创建企业帐户,为管理员的帐单和许可使用情况提供单一的可见点和管理点。'
+intro: 'With {% data variables.product.product_name %}, you can use an enterprise account to give administrators a single point of visibility and management{% if enterpriseServerVersions contains currentVersion %} for billing and license usage{% endif %}.'
redirect_from:
- /enterprise/admin/installation/about-enterprise-accounts
- /enterprise/admin/overview/about-enterprise-accounts
versions:
- enterprise-server: '*'
+ enterprise-server: '>=2.20'
+ github-ae: '*'
---
-### 关于 {% data variables.product.prodname_ghe_server %} 上的企业账户
+### 关于 {% data variables.product.product_name %} 上的企业账户
-企业帐户可用于管理多个 {% data variables.product.prodname_dotcom %} 组织和 {% data variables.product.prodname_ghe_server %} 实例。 您的企业帐户必须有操作点,如 {% data variables.product.prodname_dotcom %} 上的组织或个人帐户。 企业管理员可以管理设置和首选项,如:
+An enterprise account allows you to manage multiple organizations{% if enterpriseServerVersions contains currentVersion %} and {% data variables.product.prodname_ghe_server %} instances{% else %} on {% data variables.product.product_name %}{% endif %}. 您的企业帐户必须有操作点,如 {% data variables.product.prodname_dotcom %} 上的组织或个人帐户。 企业管理员可以管理设置和首选项,如:
-- 成员访问和管理(组织成员、外部协作者)
-- 计费和使用({% data variables.product.prodname_ghe_server %} 实例、用户许可、{% data variables.large_files.product_name_short %} 包)
-- 安全性(单点登录、双重身份验证)
-- 与 {% data variables.contact.enterprise_support %} 共享请求和支持包
+- Member access and management (organization members, outside collaborators){% if enterpriseServerVersions contains currentVersion %}
+- Billing and usage ({% data variables.product.prodname_ghe_server %} instances, user licenses, {% data variables.large_files.product_name_short %} packs){% endif %}
+- Security{% if enterpriseServerVersions contains currentVersion %}(single sign-on, two factor authentication)
+- Requests {% if enterpriseServerVersions contains currentVersion %}and support bundle sharing {% endif %}with {% data variables.contact.enterprise_support %}{% endif %}
-{% data reusables.enterprise-accounts.enterprise-accounts-billing %}
+{% if enterpriseServerVersions contains currentVersion %}{% data reusables.enterprise-accounts.enterprise-accounts-billing %} For more information about managing your {% data variables.product.prodname_ghe_cloud %} subscription, see "[Viewing the subscription and usage for your enterprise account](/articles/viewing-the-subscription-and-usage-for-your-enterprise-account)." {% endif %}For more information about managing your {% data variables.product.product_name %} billing settings, see "[Managing billing for your enterprise](/admin/overview/managing-billing-for-your-enterprise)."
+
+{% if enterpriseServerVersions contains currentVersion %}
有关 {% data variables.product.prodname_ghe_cloud %} 与 {% data variables.product.prodname_ghe_server %} 之间差异的更多信息,请参阅“[{% data variables.product.prodname_dotcom %} 的产品](/articles/githubs-products)”。 要升级至 {% data variables.product.prodname_enterprise %} 或开始使用企业帐户,请联系 {% data variables.contact.contact_enterprise_sales %}。
### 管理链接至企业帐户的 {% data variables.product.prodname_ghe_server %} 许可
{% data reusables.enterprise-accounts.admin-managing-licenses %}
+
+{% endif %}
diff --git a/translations/zh-CN/content/admin/packages/configuring-third-party-storage-for-packages.md b/translations/zh-CN/content/admin/packages/configuring-third-party-storage-for-packages.md
index 381702d2bacb..3c7b2834586f 100644
--- a/translations/zh-CN/content/admin/packages/configuring-third-party-storage-for-packages.md
+++ b/translations/zh-CN/content/admin/packages/configuring-third-party-storage-for-packages.md
@@ -13,7 +13,7 @@ versions:
{% data variables.product.prodname_ghe_server %} 上的 {% data variables.product.prodname_registry %} 使用外部 Blob 存储来存储您的软件包。 所需存储量取决于您使用 {% data variables.product.prodname_registry %} 的情况。
-目前,{% data variables.product.prodname_registry %} 支持使用 Amazon Web Services (AWS) S3 的 Blob 存储。 还支持 MinIO,但配置当前未在 {% data variables.product.product_name %} 界面中实现。 您可以按照 AWS S3 的说明使用 MinIO 进行存储,输入 MinIO 配置的类似信息。
+目前,{% data variables.product.prodname_registry %} 支持使用 Amazon Web Services (AWS) S3 的 Blob 存储。 还支持 MinIO,但配置当前未在 {% data variables.product.product_name %} 界面中实现。 您可以按照 AWS S3 的说明使用 MinIO 进行存储,输入 MinIO 配置的类似信息。 Before configuring third-party storage for {% data variables.product.prodname_registry %} on {% data variables.product.prodname_dotcom %}, you must set up a bucket with your third-party storage provider. For more information on installing and running a MinIO bucket to use with {% data variables.product.prodname_registry %}, see the "[Quickstart for configuring MinIO storage](/admin/packages/quickstart-for-configuring-minio-storage)."
为了获得最佳体验,我们建议对 {% data variables.product.prodname_registry %} 使用专用存储桶,与用于 {% data variables.product.prodname_actions %} 存储的存储桶分开。
diff --git a/translations/zh-CN/content/admin/packages/index.md b/translations/zh-CN/content/admin/packages/index.md
index 30331937754b..a063b109e858 100644
--- a/translations/zh-CN/content/admin/packages/index.md
+++ b/translations/zh-CN/content/admin/packages/index.md
@@ -10,5 +10,6 @@ versions:
{% data reusables.package_registry.packages-ghes-release-stage %}
{% link_with_intro /enabling-github-packages-for-your-enterprise %}
+{% link_with_intro /quickstart-for-configuring-minio-storage %}
{% link_with_intro /configuring-packages-support-for-your-enterprise %}
{% link_with_intro /configuring-third-party-storage-for-packages %}
diff --git a/translations/zh-CN/content/admin/packages/quickstart-for-configuring-minio-storage.md b/translations/zh-CN/content/admin/packages/quickstart-for-configuring-minio-storage.md
new file mode 100644
index 000000000000..bf6565fef27a
--- /dev/null
+++ b/translations/zh-CN/content/admin/packages/quickstart-for-configuring-minio-storage.md
@@ -0,0 +1,133 @@
+---
+title: Quickstart for configuring MinIO storage
+intro: 'Set up MinIO as a storage provider for using {% data variables.product.prodname_registry %} on your enterprise.'
+versions:
+ enterprise-server: '>=2.22'
+---
+
+{% data reusables.package_registry.packages-ghes-release-stage %}
+
+Before you can enable and configure {% data variables.product.prodname_registry %} on {% data variables.product.product_location_enterprise %}, you need to prepare your third-party storage solution.
+
+MinIO offers object storage with support for the S3 API and {% data variables.product.prodname_registry %} on your enterprise.
+
+This quickstart shows you how to set up MinIO using Docker for use with {% data variables.product.prodname_registry %} but you have other options for managing MinIO besides Docker. For more information about MinIO, see the official [MinIO docs](https://docs.min.io/).
+
+### 1. Choose a MinIO mode for your needs
+
+| MinIO mode | Optimized for | Storage infrastructure required |
+| ----------------------------------------------- | ------------------------------ | ------------------------------------ |
+| Standalone MinIO (on a single host) | Fast setup | 不适用 |
+| MinIO as a NAS gateway | NAS (Network-attached storage) | NAS devices |
+| Clustered MinIO (also called Distributed MinIO) | Data security | Storage servers running in a cluster |
+
+For more information about your options, see the official [MinIO docs](https://docs.min.io/).
+
+### 2. Install, run, and sign in to MinIO
+
+1. Set up your preferred environment variables for MinIO.
+
+ These examples use `MINIO_DIR`:
+ ```shell
+ $ export MINIO_DIR=$(pwd)/minio
+ $ mkdir -p $MINIO_DIR
+ ```
+
+2. Install MinIO.
+
+ ```shell
+ $ docker pull minio/minio
+ ```
+ For more information, see the official "[MinIO Quickstart Guide](https://docs.min.io/docs/minio-quickstart-guide)."
+
+3. Sign in to MinIO using your MinIO access key and secret.
+
+ {% linux %}
+ ```shell
+ $ export MINIO_ACCESS_KEY=$(cat /dev/urandom | tr -dc 'a-zA-Z0-9' | fold -w 32 | head -n 1)
+ # this one is actually a secret, so careful
+ $ export MINIO_SECRET_KEY=$(cat /dev/urandom | tr -dc 'a-zA-Z0-9' | fold -w 32 | head -n 1)
+ ```
+ {% endlinux %}
+
+ {% mac %}
+ ```shell
+ $ export MINIO_ACCESS_KEY=$(cat /dev/urandom | LC_CTYPE=C tr -dc 'a-zA-Z0-9' | fold -w 32 | head -n 1)
+ # this one is actually a secret, so careful
+ $ export MINIO_SECRET_KEY=$(cat /dev/urandom | LC_CTYPE=C tr -dc 'a-zA-Z0-9' | fold -w 32 | head -n 1)
+ ```
+ {% endmac %}
+
+ You can access your MinIO keys using the environment variables:
+
+ ```shell
+ $ echo $MINIO_ACCESS_KEY
+ $ echo $MINIO_SECRET_KEY
+ ```
+
+4. Run MinIO in your chosen mode.
+
+ * Run MinIO using Docker on a single host:
+
+ ```shell
+ $ docker run -p 9000:9000 \
+ -v $MINIO_DIR:/data \
+ -e "MINIO_ACCESS_KEY=$MINIO_ACCESS_KEY" \
+ -e "MINIO_SECRET_KEY=$MINIO_SECRET_KEY" \
+ minio/minio server /data
+ ```
+
+ For more information, see "[MinIO Docker Quickstart guide](https://docs.min.io/docs/minio-docker-quickstart-guide.html)."
+
+ * Run MinIO using Docker as a NAS gateway:
+
+ This setup is useful for deployments where there is already a NAS you want to use as the backup storage for {% data variables.product.prodname_registry %}.
+
+ ```shell
+ $ docker run -p 9000:9000 \
+ -v $MINIO_DIR:/data \
+ -e "MINIO_ACCESS_KEY=$MINIO_ACCESS_KEY" \
+ -e "MINIO_SECRET_KEY=$MINIO_SECRET_KEY" \
+ minio/minio gateway nas /data
+ ```
+
+ For more information, see "[MinIO Gateway for NAS](https://docs.min.io/docs/minio-gateway-for-nas.html)."
+
+ * Run MinIO using Docker as a cluster. This MinIO deployment uses several hosts and MinIO's erasure coding for the strongest data protection. To run MinIO in a cluster mode, see the "[Distributed MinIO Quickstart Guide](https://docs.min.io/docs/distributed-minio-quickstart-guide.html).
+
+### 3. Create your MinIO bucket for {% data variables.product.prodname_registry %}
+
+1. Install the MinIO client.
+
+ ```shell
+ $ docker pull minio/mc
+ ```
+
+2. Create a bucket with a host URL that {% data variables.product.prodname_ghe_server %} can access.
+
+ * Local deployments example:
+
+ ```shell
+ $ export MC_HOST_minio="http://${MINIO_ACCESS_KEY}:${MINIO_SECRET_KEY} @localhost:9000"
+ $ docker run minio/mc BUCKET-NAME
+ ```
+
+ This example can be used for MinIO standalone or MinIO as a NAS gateway.
+
+ * Clustered deployments example:
+
+ ```shell
+ $ export MC_HOST_minio="http://${MINIO_ACCESS_KEY}:${MINIO_SECRET_KEY} @minioclustername.example.com:9000"
+ $ docker run minio/mc mb packages
+ ```
+
+
+### 后续步骤
+
+To finish configuring storage for {% data variables.product.prodname_registry %}, you'll need to copy the MinIO storage URL:
+
+ ```
+ echo "http://${MINIO_ACCESS_KEY}:${MINIO_SECRET_KEY}@minioclustername.example.com:9000"
+ ```
+
+For the next steps, see "[Configuring third-party storage for packages](/admin/packages/configuring-third-party-storage-for-packages)."
\ No newline at end of file
diff --git a/translations/zh-CN/content/admin/policies/creating-a-pre-receive-hook-environment.md b/translations/zh-CN/content/admin/policies/creating-a-pre-receive-hook-environment.md
index 073aa199c2ee..e1adf7025dcc 100644
--- a/translations/zh-CN/content/admin/policies/creating-a-pre-receive-hook-environment.md
+++ b/translations/zh-CN/content/admin/policies/creating-a-pre-receive-hook-environment.md
@@ -21,10 +21,10 @@ versions:
{% data reusables.linux.ensure-docker %}
2. 创建包含此信息的文件 `Dockerfile.alpine-3.3`:
- ```
- FROM gliderlabs/alpine:3.3
- RUN apk add --no-cache git bash
- ```
+ ```
+ FROM gliderlabs/alpine:3.3
+ RUN apk add --no-cache git bash
+ ```
3. 从包含 `Dockerfile.dev` 的工作目录中,构建一个镜像:
```shell
@@ -36,37 +36,37 @@ versions:
> ---> Using cache
> ---> 0250ab3be9c5
> Successfully built 0250ab3be9c5
- ```
+ ```
4. 创建一个容器:
```shell
$ docker create --name pre-receive.alpine-3.3 pre-receive.alpine-3.3 /bin/true
- ```
+ ```
5. 将 Docker 容器导出到 `gzip` 压缩的 `tar` 文件:
```shell
$ docker export pre-receive.alpine-3.3 | gzip > alpine-3.3.tar.gz
- ```
+ ```
- 此文件 `alpine-3.3.tar.gz` 已准备好上传到 {% data variables.product.prodname_ghe_server %} 设备。
+ 此文件 `alpine-3.3.tar.gz` 已准备好上传到 {% data variables.product.prodname_ghe_server %} 设备。
### 使用 chroot 创建预接收挂钩环境
1. 创建 Linux `chroot` 环境。
2. 创建 `chroot` 目录的 `gzip` 压缩 `tar` 文件:
- ```shell
- $ cd /path/to/chroot
- $ tar -czf /path/to/pre-receive-environment.tar.gz .
+ ```shell
+ $ cd /path/to/chroot
+ $ tar -czf /path/to/pre-receive-environment.tar.gz .
```
- {% note %}
+ {% note %}
- **注意:**
- - 不要在 tar 存档中包含文件的主目录路径,如 `/path/to/chroot`。
- - `/bin/sh` 必须存在并且可执行,作为 chroot 环境的入口点。
- - 与传统的 chroot 不同,预接收挂钩的 chroot 环境不需要 `dev` 目录。
+ **注意:**
+ - 不要在 tar 存档中包含文件的主目录路径,如 `/path/to/chroot`。
+ - `/bin/sh` 必须存在并且可执行,作为 chroot 环境的入口点。
+ - 与传统的 chroot 不同,预接收挂钩的 chroot 环境不需要 `dev` 目录。
- {% endnote %}
+ {% endnote %}
关于创建 chroot 环境的更多信息,请参阅 *Debian Wiki* 中的“[Chroot](https://wiki.debian.org/chroot)”、*Ubuntu 社区帮助 Wiki* 中的“[BasicChroot](https://help.ubuntu.com/community/BasicChroot)”,或者 *Alpine Linux Wiki* 中的“[在 chroot 中安装 Alpine Linux](http://wiki.alpinelinux.org/wiki/Installing_Alpine_Linux_in_a_chroot)”。
@@ -89,4 +89,4 @@ versions:
```shell
admin@ghe-host:~$ ghe-hook-env-create AlpineTestEnv /home/admin/alpine-3.3.tar.gz
> Pre-receive hook environment 'AlpineTestEnv' (2) has been created.
- ```
+ ```
diff --git a/translations/zh-CN/content/admin/policies/creating-a-pre-receive-hook-script.md b/translations/zh-CN/content/admin/policies/creating-a-pre-receive-hook-script.md
index 312242776fef..621386099b4e 100644
--- a/translations/zh-CN/content/admin/policies/creating-a-pre-receive-hook-script.md
+++ b/translations/zh-CN/content/admin/policies/creating-a-pre-receive-hook-script.md
@@ -70,19 +70,19 @@ versions:
```shell
$ sudo chmod +x SCRIPT_FILE.sh
- ```
- 对于 Windows 用户,确保脚本具有执行权限:
+ ```
+ 对于 Windows 用户,确保脚本具有执行权限:
- ```shell
- git update-index --chmod=+x SCRIPT_FILE.sh
- ```
+ ```shell
+ git update-index --chmod=+x SCRIPT_FILE.sh
+ ```
2. 提交并推送到 {% data variables.product.prodname_ghe_server %} 实例上指定的预接收挂钩仓库。
```shell
$ git commit -m "YOUR COMMIT MESSAGE"
$ git push
- ```
+ ```
3. 在 {% data variables.product.prodname_ghe_server %} 实例上[创建预接收挂钩](/enterprise/{{ currentVersion }}/admin/guides/developer-workflow/managing-pre-receive-hooks-on-the-github-enterprise-server-appliance/#creating-pre-receive-hooks)。
@@ -93,40 +93,40 @@ versions:
2. 创建一个名为 `Dockerfile.dev` 的文件,其中包含:
- ```
- FROM gliderlabs/alpine:3.3
- RUN \
- apk add --no-cache git openssh bash && \
- ssh-keygen -A && \
- sed -i "s/#AuthorizedKeysFile/AuthorizedKeysFile/g" /etc/ssh/sshd_config && \
- adduser git -D -G root -h /home/git -s /bin/bash && \
- passwd -d git && \
- su git -c "mkdir /home/git/.ssh && \
- ssh-keygen -t ed25519 -f /home/git/.ssh/id_ed25519 -P '' && \
- mv /home/git/.ssh/id_ed25519.pub /home/git/.ssh/authorized_keys && \
- mkdir /home/git/test.git && \
- git --bare init /home/git/test.git"
-
- VOLUME ["/home/git/.ssh", "/home/git/test.git/hooks"]
- WORKDIR /home/git
-
- CMD ["/usr/sbin/sshd", "-D"]
- ```
+ ```
+ FROM gliderlabs/alpine:3.3
+ RUN \
+ apk add --no-cache git openssh bash && \
+ ssh-keygen -A && \
+ sed -i "s/#AuthorizedKeysFile/AuthorizedKeysFile/g" /etc/ssh/sshd_config && \
+ adduser git -D -G root -h /home/git -s /bin/bash && \
+ passwd -d git && \
+ su git -c "mkdir /home/git/.ssh && \
+ ssh-keygen -t ed25519 -f /home/git/.ssh/id_ed25519 -P '' && \
+ mv /home/git/.ssh/id_ed25519.pub /home/git/.ssh/authorized_keys && \
+ mkdir /home/git/test.git && \
+ git --bare init /home/git/test.git"
+
+ VOLUME ["/home/git/.ssh", "/home/git/test.git/hooks"]
+ WORKDIR /home/git
+
+ CMD ["/usr/sbin/sshd", "-D"]
+ ```
3. 创建一个名为 `always_reject.sh` 的测试预接收脚本。 此示例脚本将拒绝所有推送,这对于锁定仓库非常有用:
- ```
- #!/usr/bin/env bash
+ ```
+ #!/usr/bin/env bash
- echo "error: rejecting all pushes"
- exit 1
- ```
+ echo "error: rejecting all pushes"
+ exit 1
+ ```
4. 确保 `always_reject.sh` 脚本具有执行权限:
```shell
$ chmod +x always_reject.sh
- ```
+ ```
5. 从包含 `Dockerfile.dev` 的目录中,构建一个镜像:
@@ -149,32 +149,32 @@ versions:
....truncated output....
> Initialized empty Git repository in /home/git/test.git/
> Successfully built dd8610c24f82
- ```
+ ```
6. 运行包含生成的 SSH 密钥的数据容器:
```shell
$ docker run --name data pre-receive.dev /bin/true
- ```
+ ```
7. 将测试预接收挂钩 `always_reject.sh` 复制到数据容器中:
```shell
$ docker cp always_reject.sh data:/home/git/test.git/hooks/pre-receive
- ```
+ ```
8. 启动一个运行 `sshd` 的应用程序容器并执行挂钩。 记下返回的容器 ID:
```shell
$ docker run -d -p 52311:22 --volumes-from data pre-receive.dev
> 7f888bc700b8d23405dbcaf039e6c71d486793cad7d8ae4dd184f4a47000bc58
- ```
+ ```
9. 将生成的 SSH 密钥从数据容器复制到本地计算机:
```shell
$ docker cp data:/home/git/.ssh/id_ed25519 .
- ```
+ ```
10. 修改远程测试仓库并将其推送到 Docker 容器中的 `test.git` 仓库。 此示例使用了 `git@github.com:octocat/Hello-World.git`,但您可以使用想要的任何仓库。 此示例假定您的本地计算机 (127.0.0.1) 绑定了端口 52311,但如果 docker 在远程计算机上运行,则可以使用不同的 IP 地址。
@@ -193,9 +193,9 @@ versions:
> To git@192.168.99.100:test.git
> ! [remote rejected] main -> main (pre-receive hook declined)
> error: failed to push some refs to 'git@192.168.99.100:test.git'
- ```
+ ```
- 请注意,在执行预接收挂钩并回显脚本中的输出后,将拒绝推送。
+ 请注意,在执行预接收挂钩并回显脚本中的输出后,将拒绝推送。
### 延伸阅读
- 来自 *Pro Git 网站*的“[自定义 Git - Git 强制实施策略示例](https://git-scm.com/book/en/v2/Customizing-Git-An-Example-Git-Enforced-Policy)”
diff --git a/translations/zh-CN/content/admin/policies/enforcing-repository-management-policies-in-your-enterprise.md b/translations/zh-CN/content/admin/policies/enforcing-repository-management-policies-in-your-enterprise.md
index f92a91da7225..f3f1e3cc1984 100644
--- a/translations/zh-CN/content/admin/policies/enforcing-repository-management-policies-in-your-enterprise.md
+++ b/translations/zh-CN/content/admin/policies/enforcing-repository-management-policies-in-your-enterprise.md
@@ -34,7 +34,7 @@ versions:
每次有人在您的企业上创建新仓库时,此人必须选择仓库的可见性。 当您配置企业的默认可见性设置时,需要选择默认可见性。 有关仓库可见性的更多信息,请参阅“[关于仓库可见性](/github/creating-cloning-and-archiving-repositories/about-repository-visibility)。”
-如果站点管理员不允许成员创建某种类型的仓库,成员将无法创建此类仓库,即使可见性设置默认为此类型。 更多信息请参阅“[设置仓库创建策略](#setting-a-policy-for-repository-creation)”。
+If an enterprise owner disallows members from creating certain types of repositories, members will not be able to create that type of repository even if the visibility setting defaults to that type. 更多信息请参阅“[设置仓库创建策略](#setting-a-policy-for-repository-creation)”。
{% data reusables.enterprise-accounts.access-enterprise %}
{% if currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
@@ -49,9 +49,9 @@ versions:
### 设置有关更改仓库可见性的策略
-当您阻止成员更改仓库可见性时,只有站点管理员可以将公共仓库设置为私有或者将私有仓库设置为公共。
+When you prevent members from changing repository visibility, only enterprise owners can change the visibility of a repository.
-如果站点管理员仅允许组织所有者创建仓库,成员将无法更改仓库可见性。 如果站点管理员只允许成员创建私有仓库,则成员只能将仓库从公共更改为私有。 更多信息请参阅“[设置仓库创建策略](#setting-a-policy-for-repository-creation)”。
+If an enterprise owner has restricted repository creation to organization owners only, then members will not be able to change repository visibility. If an enterprise owner has restricted member repository creation to private repositories only, then members will only be able to change the visibility of a repository to private. 更多信息请参阅“[设置仓库创建策略](#setting-a-policy-for-repository-creation)”。
{% data reusables.enterprise-accounts.access-enterprise %}
{% data reusables.enterprise-accounts.policies-tab %}
@@ -75,6 +75,15 @@ versions:
6. 在“Repository creation(仓库创建)”下,使用下拉菜单并选择策略。 
{% endif %}
+### 实施有关复刻私有或内部仓库的策略
+
+Across all organizations owned by your enterprise, you can allow people with access to a private or internal repository to fork the repository, never allow forking of private or internal repositories, or allow owners to administer the setting on the organization level.
+
+{% data reusables.enterprise-accounts.access-enterprise %}
+{% data reusables.enterprise-accounts.policies-tab %}
+3. 在 **Repository policies(仓库策略)**选项卡中的“Repository forking(仓库复刻)”下,审查有关更改设置的信息。 {% data reusables.enterprise-accounts.view-current-policy-config-orgs %}
+4. 在“Repository forking(仓库复刻)”下,使用下拉菜单并选择策略。 
+
### 设置仓库删除和转移的策略
{% data reusables.enterprise-accounts.access-enterprise %}
@@ -166,6 +175,8 @@ versions:
- **Block to the default branch** 来仅阻止对默认分支进行强制推送。 
6. 可以视情况选择 **Enforce on all repositories** 来覆盖仓库特定的设置。 注意,这**不**会覆盖企业范围的策略。 
+{% if enterpriseServerVersions contains currentVersion %}
+
### 配置匿名 Git 读取访问
{% data reusables.enterprise_user_management.disclaimer-for-git-read-access %}
@@ -192,7 +203,6 @@ versions:
4. 在“Anonymous Git read access”下,使用下列菜单并单击 **Enabled**。 
3. 或者,如果要阻止仓库管理员为企业上的所有仓库更改匿名 Git 读取权限设置,请选择 **Prevent repository admins from changing anonymous Git read access**。 
-{% if enterpriseServerVersions contains currentVersion %}
#### 设置特定仓库的匿名 Git 读取访问
{% data reusables.enterprise_site_admin_settings.access-settings %}
@@ -203,6 +213,7 @@ versions:
6. 在“Danger Zone”下的“Enable Anonymous Git read access”旁,请单击 **Enable**。 
7. 审查更改。 要确认,请单击 **Yes, enable anonymous Git read access(是,启用匿名 Git 读取权限)**。 
8. 或者,如果要阻止仓库管理员为此仓库更改设置,请选择 **Prevent repository admins from changing anonymous Git read access**。 
+
{% endif %}
{% if currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
diff --git a/translations/zh-CN/content/admin/release-notes.md b/translations/zh-CN/content/admin/release-notes.md
new file mode 100644
index 000000000000..435693295c0c
--- /dev/null
+++ b/translations/zh-CN/content/admin/release-notes.md
@@ -0,0 +1,8 @@
+---
+title: 发行说明
+intro: The release notes for {{ allVersions[currentVersion].versionTitle }}.
+layout: release-notes
+versions:
+ enterprise-server: '*'
+---
+
diff --git a/translations/zh-CN/content/admin/user-management/audited-actions.md b/translations/zh-CN/content/admin/user-management/audited-actions.md
index 79f99394740b..d5d9ad32ee1a 100644
--- a/translations/zh-CN/content/admin/user-management/audited-actions.md
+++ b/translations/zh-CN/content/admin/user-management/audited-actions.md
@@ -36,12 +36,12 @@ versions:
#### 企业配置设置
-| 名称 | 描述 |
-| -------------------------------------------------------:| ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
-| `business.update_member_repository_creation_permission` | 站点管理员限制在企业中的组织中创建仓库。 更多信息请参阅“[在企业中实施仓库管理策略](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#setting-a-policy-for-repository-creation)”。 |
-| `business.clear_members_can_create_repos` | 站点管理员取消了对在企业中的组织中创建仓库的限制。 更多信息请参阅“[在企业中实施仓库管理策略](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#setting-a-policy-for-repository-creation)”。 |
-| `enterprise.config.lock_anonymous_git_access` | 站点管理员锁定匿名 Git 读取权限,以防止仓库管理员更改该企业中仓库的现有匿名 Git 读取权限设置。 更多信息请参阅“[在企业中实施仓库管理策略](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#configuring-anonymous-git-read-access)”。 |
-| `enterprise.config.unlock_anonymous_git_access` | 站点管理员解锁匿名 Git 读取权限,以允许仓库管理员更改该企业中仓库的现有匿名 Git 读取权限设置。 更多信息请参阅“[在企业中实施仓库管理策略](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#configuring-anonymous-git-read-access)”。 |
+| 名称 | 描述 |
+| -------------------------------------------------------:| --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `business.update_member_repository_creation_permission` | 站点管理员限制在企业中的组织中创建仓库。 更多信息请参阅“[在企业中实施仓库管理策略](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#setting-a-policy-for-repository-creation)”。 |
+| `business.clear_members_can_create_repos` | 站点管理员取消了对在企业中的组织中创建仓库的限制。 For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#setting-a-policy-for-repository-creation)."{% if enterpriseServerVersions contains currentVersion %}
+| `enterprise.config.lock_anonymous_git_access` | 站点管理员锁定匿名 Git 读取权限,以防止仓库管理员更改该企业中仓库的现有匿名 Git 读取权限设置。 更多信息请参阅“[在企业中实施仓库管理策略](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#configuring-anonymous-git-read-access)”。 |
+| `enterprise.config.unlock_anonymous_git_access` | 站点管理员解锁匿名 Git 读取权限,以允许仓库管理员更改该企业中仓库的现有匿名 Git 读取权限设置。 For more information, see "[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#configuring-anonymous-git-read-access)."{% endif %}
#### 议题和拉取请求
@@ -77,23 +77,23 @@ versions:
#### 仓库
-| 名称 | 描述 |
-| ------------------------------------------:| ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| `repo.access` | 已将私有仓库设为公共,或者已将公共仓库设为私有。 |
-| `repo.archive` | 已存档仓库。 更多信息请参阅“[存档 {% data variables.product.prodname_dotcom %} 仓库](/github/creating-cloning-and-archiving-repositories/archiving-a-github-repository)”。 |
-| `repo.add_member` | 已向仓库添加协作者。 |
-| `repo.config` | 站点管理员已阻止强制推送。 更多信息请参阅“[阻止对仓库进行强制推送](/enterprise/{{ currentVersion }}/admin/guides/developer-workflow/blocking-force-pushes-to-a-repository/)”。 |
-| `repo.create` | 已创建仓库。 |
-| `repo.destroy` | 已删除仓库。 |
-| `repo.remove_member` | 已从仓库中移除协作者。 |
-| `repo.rename` | 已重命名仓库。 |
-| `repo.transfer` | 用户已接受接收传输仓库的请求。 |
-| `repo.transfer_start` | 用户已发送向另一用户或组织传输仓库的请求。 |
-| `repo.unarchive` | 已取消存档仓库。 更多信息请参阅“[存档 {% data variables.product.prodname_dotcom %} 仓库](/github/creating-cloning-and-archiving-repositories/archiving-a-github-repository)”。 |
-| `repo.config.disable_anonymous_git_access` | 已为公共仓库禁用匿名 Git 读取权限。 更多信息请参阅“[为仓库启用匿名 Git 读取权限](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)。” |
-| `repo.config.enable_anonymous_git_access` | 已为公共仓库启用匿名 Git 读取权限。 更多信息请参阅“[为仓库启用匿名 Git 读取权限](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)。” |
-| `repo.config.lock_anonymous_git_access` | 已锁定仓库的匿名 Git 读取权限设置,阻止仓库管理员更改(启用或禁用)此设置。 更多信息请参阅“[阻止用户更改匿名 Git 读取权限](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)”。 |
-| `repo.config.unlock_anonymous_git_access` | 已解锁仓库的匿名 Git 读取权限设置,允许仓库管理员更改(启用或禁用)此设置。 更多信息请参阅“[阻止用户更改匿名 Git 读取权限](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)”。 |
+| 名称 | 描述 |
+| ------------------------------------------:| ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `repo.access` | The visibility of a repository changed to private{% if enterpriseServerVersions contains currentVersion %}, public,{% endif %} or internal. |
+| `repo.archive` | 已存档仓库。 更多信息请参阅“[存档 {% data variables.product.prodname_dotcom %} 仓库](/github/creating-cloning-and-archiving-repositories/archiving-a-github-repository)”。 |
+| `repo.add_member` | 已向仓库添加协作者。 |
+| `repo.config` | 站点管理员已阻止强制推送。 更多信息请参阅“[阻止对仓库进行强制推送](/enterprise/{{ currentVersion }}/admin/guides/developer-workflow/blocking-force-pushes-to-a-repository/)”。 |
+| `repo.create` | 已创建仓库。 |
+| `repo.destroy` | 已删除仓库。 |
+| `repo.remove_member` | 已从仓库中移除协作者。 |
+| `repo.rename` | 已重命名仓库。 |
+| `repo.transfer` | 用户已接受接收传输仓库的请求。 |
+| `repo.transfer_start` | 用户已发送向另一用户或组织传输仓库的请求。 |
+| `repo.unarchive` | 已取消存档仓库。 For more information, see "[Archiving a {% data variables.product.prodname_dotcom %} repository](/github/creating-cloning-and-archiving-repositories/archiving-a-github-repository)."{% if enterpriseServerVersions contains currentVersion %}
+| `repo.config.disable_anonymous_git_access` | Anonymous Git read access is disabled for a repository. 更多信息请参阅“[为仓库启用匿名 Git 读取权限](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)。” |
+| `repo.config.enable_anonymous_git_access` | Anonymous Git read access is enabled for a repository. 更多信息请参阅“[为仓库启用匿名 Git 读取权限](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)。” |
+| `repo.config.lock_anonymous_git_access` | 已锁定仓库的匿名 Git 读取权限设置,阻止仓库管理员更改(启用或禁用)此设置。 更多信息请参阅“[阻止用户更改匿名 Git 读取权限](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)”。 |
+| `repo.config.unlock_anonymous_git_access` | 已解锁仓库的匿名 Git 读取权限设置,允许仓库管理员更改(启用或禁用)此设置。 For more information, see "[Preventing users from changing anonymous Git read access](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)."{% endif %}
#### 站点管理员工具
diff --git a/translations/zh-CN/content/admin/user-management/managing-organizations-in-your-enterprise.md b/translations/zh-CN/content/admin/user-management/managing-organizations-in-your-enterprise.md
index 2e44ecf6e06c..294985b047b1 100644
--- a/translations/zh-CN/content/admin/user-management/managing-organizations-in-your-enterprise.md
+++ b/translations/zh-CN/content/admin/user-management/managing-organizations-in-your-enterprise.md
@@ -5,7 +5,7 @@ redirect_from:
- /enterprise/admin/categories/admin-bootcamp/
- /enterprise/admin/user-management/organizations-and-teams
- /enterprise/admin/user-management/managing-organizations-in-your-enterprise
-intro: '组织适合在您的公司内创建不同的用户组,例如部门或参与相似项目的组。 属于某个组织的公共仓库也可供其他组织的用户使用,但私有仓库仅供该组织的成员使用。'
+intro: '组织适合在您的公司内创建不同的用户组,例如部门或参与相似项目的组。 {% if currentVersion == "github-ae@latest" %}Internal{% else %}Public and internal{% endif %} repositories that belong to an organization are accessible to users in other organizations, while private repositories are inaccessible to anyone but members of the organization that are granted access.'
mapTopic: true
versions:
enterprise-server: '*'
diff --git a/translations/zh-CN/content/developers/apps/about-apps.md b/translations/zh-CN/content/developers/apps/about-apps.md
index 0518979d17f7..b2affb42629f 100644
--- a/translations/zh-CN/content/developers/apps/about-apps.md
+++ b/translations/zh-CN/content/developers/apps/about-apps.md
@@ -1,6 +1,6 @@
---
title: 关于应用程序
-intro: 'You can build integrations with the {% data variables.product.prodname_dotcom %} APIs to add flexibility and reduce friction in your own workflow.{% if currentVersion == "free-pro-team@latest" %} You can also share integrations with others on [{% data variables.product.prodname_marketplace %}](https://github.com/marketplace).{% endif %}'
+intro: '您可以构建与 {% data variables.product.prodname_dotcom %} API 的集成来增加灵活性并减少自己工作流程中的摩擦。{% if currentVersion == "free-pro-team@latest" %} 您也可以与 [{% data variables.product.prodname_marketplace %}](https://github.com/marketplace) 上的其他API 集成。{% endif %}'
redirect_from:
- /apps/building-integrations/setting-up-a-new-integration/
- /apps/building-integrations/
@@ -12,37 +12,37 @@ versions:
github-ae: '*'
---
-Apps on {% data variables.product.prodname_dotcom %} allow you to automate and improve your workflow. You can build apps to improve your workflow.{% if currentVersion == "free-pro-team@latest" %} You can also share or sell apps in [{% data variables.product.prodname_marketplace %}](https://github.com/marketplace). To learn how to list an app on {% data variables.product.prodname_marketplace %}, see "[Getting started with GitHub Marketplace](/marketplace/getting-started/)."{% endif %}
+{% data variables.product.prodname_dotcom %} 上的应用程序允许您自动化并改进工作流程。 您可以构建应用程序来改进工作流程。{% if currentversion == "free proteam@latest" %} 您也可以在 [{% data variables.product.prodname_marketplace %}](https://github.com/marketplace) 中分享或销售应用程序。 要了解如何在 {% data variables.product.prodname_marketplace %} 上列出应用程序,请参阅“[GitHub Marketplace 使用入门](/marketplace/getting-started/)”。{% endif %}
-{% data reusables.marketplace.github_apps_preferred %}, but GitHub supports both {% data variables.product.prodname_oauth_app %}s and {% data variables.product.prodname_github_apps %}. For information on choosing a type of app, see "[About apps](/apps/about-apps/)" and "[Differences between apps](/apps/differences-between-apps/)."
+{% data reusables.marketplace.github_apps_preferred %},但 GitHub 支持 {% data variables.product.prodname_oauth_app %} 和 {% data variables.product.prodname_github_apps %}。 有关选择应用程序类型的信息,请参阅“[关于应用程序](/apps/about-apps/)”和“[应用程序之间的差异](/apps/differences-between-apps/)”。
{% data reusables.apps.general-apps-restrictions %}
-For a walkthrough of the process of building a {% data variables.product.prodname_github_app %}, see "[Building Your First {% data variables.product.prodname_github_app %}](/apps/building-your-first-github-app)."
+有关构建 {% data variables.product.prodname_github_app %} 的过程演练,请参阅“[构建第一个 {% data variables.product.prodname_github_app %}](/apps/building-your-first-github-app)”。
### 关于 {% data variables.product.prodname_github_apps %}
-{% data variables.product.prodname_github_apps %} are first-class actors within GitHub. A {% data variables.product.prodname_github_app %} acts on its own behalf, taking actions via the API directly using its own identity, which means you don't need to maintain a bot or service account as a separate user.
+{% data variables.product.prodname_github_apps %} 是 GitHub 中的一流产品。 {% data variables.product.prodname_github_app %} 应用程序以自己的名义运行,通过 API 直接使用自己的身份进行操作,这意味着您无需作为独立用户维护自动程序或服务帐户。
-{% data variables.product.prodname_github_apps %} can be installed directly on organizations and user accounts and granted access to specific repositories. 它们拥有内置 web 挂钩和狭窄的特定权限。 When you set up your {% data variables.product.prodname_github_app %}, you can select the repositories you want it to access. 例如,您可以设置一个名为 `MyGitHub` 的应用程序,允许它在 `octocat` 仓库且_仅_在 `octocat` 仓库写入议题。 To install a {% data variables.product.prodname_github_app %}, you must be an organization owner or have admin permissions in a repository.
+{% data variables.product.prodname_github_apps %} 可以直接安装在组织和用户帐户上,并获得对特定仓库的访问权限。 它们拥有内置 web 挂钩和狭窄的特定权限。 设置 {% data variables.product.prodname_github_app %}时,可以选择希望它访问的仓库。 例如,您可以设置一个名为 `MyGitHub` 的应用程序,允许它在 `octocat` 仓库且_仅_在 `octocat` 仓库写入议题。 要安装 {% data variables.product.prodname_github_app %},您必须是组织所有者或在仓库中具有管理员权限。
{% data reusables.apps.app_manager_role %}
-{% data variables.product.prodname_github_apps %} are applications that need to be hosted somewhere. For step-by-step instructions that cover servers and hosting, see "[Building Your First {% data variables.product.prodname_github_app %}](/apps/building-your-first-github-app)."
+{% data variables.product.prodname_github_apps %} 是需要托管在某处的应用程序。 有关涵盖服务器和托管服务的分步说明,请参阅“[构建第一个 {% data variables.product.prodname_github_app %}](/apps/building-your-first-github-app)。”
-To improve your workflow, you can create a {% data variables.product.prodname_github_app %} that contains multiple scripts or an entire application, and then connect that app to many other tools. For example, you can connect {% data variables.product.prodname_github_apps %} to GitHub, Slack, other in-house apps you may have, email programs, or other APIs.
+要改进自己的工作流程,可以创建一个包含多个脚本或整个应用程序的 {% data variables.product.prodname_github_app %},然后将此应用程序连接至许多其他工具。 例如,您可以将 {% data variables.product.prodname_github_apps %} 连接至 GitHub、Slack、自己可能拥有的其他内部应用程序、电子邮件程序或其他 API。
-Keep these ideas in mind when creating {% data variables.product.prodname_github_apps %}:
+创建 {% data variables.product.prodname_github_apps %} 时,请记住以下方法:
{% if currentVersion == "free-pro-team@latest" %}
* {% data reusables.apps.maximum-github-apps-allowed %} {% endif %}
-* A {% data variables.product.prodname_github_app %} should take actions independent of a user (unless the app is using a [user-to-server](/apps/building-github-apps/identifying-and-authorizing-users-for-github-apps#user-to-server-requests) token). {% data reusables.apps.expiring_user_authorization_tokens %}
+* {% data variables.product.prodname_github_app %} 的操作应独立于用户(除非此应用程序使用[用户至服务器](/apps/building-github-apps/identifying-and-authorizing-users-for-github-apps#user-to-server-requests)令牌)。 {% data reusables.apps.expiring_user_authorization_tokens %}
-* Make sure the {% data variables.product.prodname_github_app %} integrates with specific repositories.
-* The {% data variables.product.prodname_github_app %} should connect to a personal account or an organization.
-* Don't expect the {% data variables.product.prodname_github_app %} to know and do everything a user can.
-* Don't use a {% data variables.product.prodname_github_app %} if you just need a "Login with GitHub" service. But a {% data variables.product.prodname_github_app %} can use a [user identification flow](/apps/building-github-apps/identifying-and-authorizing-users-for-github-apps/) to log users in _and_ do other things.
-* Don't build a {% data variables.product.prodname_github_app %} if you _only_ want to act as a GitHub user and do everything that user can do.{% if currentVersion == "free-pro-team@latest" %}
+* 请确保 {% data variables.product.prodname_github_app %} 与特定仓库集成。
+* {% data variables.product.prodname_github_app %} 应该连接到个人帐户或组织。
+* 不要指望 {% data variables.product.prodname_github_app %} 知道并尽其所能。
+* 如果您只需要“使用GitHub登录”服务,请不要使用 {% data variables.product.prodname_github_app %}。 但是,{% data variables.product.prodname_github_app %} 可以使用[用户识别流程](/apps/building-github-apps/identifying-and-authorizing-users-for-github-apps/)登录用户账户_和_执行其他操作。
+* 如果您_只_想作为 GitHub 用户并做用户可以执行的所有操作,请不要构建 {% data variables.product.prodname_github_app %}。{% if currentVersion == "free-pro-team@latest" %}
* {% data reusables.apps.general-apps-restrictions %}{% endif %}
To begin developing {% data variables.product.prodname_github_apps %}, start with "[Creating a {% data variables.product.prodname_github_app %}](/apps/building-github-apps/creating-a-github-app/)."{% if currentVersion == "free-pro-team@latest" %} To learn how to use {% data variables.product.prodname_github_app %} Manifests, which allow people to create preconfigured {% data variables.product.prodname_github_apps %}, see "[Creating {% data variables.product.prodname_github_apps %} from a manifest](/apps/building-github-apps/creating-github-apps-from-a-manifest/)."{% endif %}
diff --git a/translations/zh-CN/content/developers/apps/authorizing-oauth-apps.md b/translations/zh-CN/content/developers/apps/authorizing-oauth-apps.md
index 4fc1213de322..42b9da91d5e5 100644
--- a/translations/zh-CN/content/developers/apps/authorizing-oauth-apps.md
+++ b/translations/zh-CN/content/developers/apps/authorizing-oauth-apps.md
@@ -114,11 +114,13 @@ curl -H "Authorization: token OAUTH-TOKEN" {% data variables.product.api_url_pre
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
### Device flow
+{% if currentVersion ver_lt "enterprise-server@3.1" %}
{% note %}
-**Note:** The device flow is in public beta and subject to change.{% if currentVersion == "free-pro-team@latest" %} To enable this beta feature, see "[Activating beta features for apps](/developers/apps/activating-beta-features-for-apps)."{% endif %}
+**Note:** The device flow is in public beta and subject to change.
{% endnote %}
+{% endif %}
The device flow allows you to authorize users for a headless app, such as a CLI tool or Git credential manager.
diff --git a/translations/zh-CN/content/developers/apps/identifying-and-authorizing-users-for-github-apps.md b/translations/zh-CN/content/developers/apps/identifying-and-authorizing-users-for-github-apps.md
index c45cf929022e..314ead212d18 100644
--- a/translations/zh-CN/content/developers/apps/identifying-and-authorizing-users-for-github-apps.md
+++ b/translations/zh-CN/content/developers/apps/identifying-and-authorizing-users-for-github-apps.md
@@ -123,11 +123,13 @@ curl -H "Authorization: token OAUTH-TOKEN" {% data variables.product.api_url_pre
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
### Device flow
+{% if currentVersion ver_lt "enterprise-server@3.1" %}
{% note %}
-**Note:** The device flow is in public beta and subject to change.{% if currentVersion == "free-pro-team@latest" %} To enable this beta feature, see "[Activating beta features for apps](/developers/apps/activating-beta-features-for-apps)."{% endif %}
+**Note:** The device flow is in public beta and subject to change.
{% endnote %}
+{% endif %}
The device flow allows you to authorize users for a headless app, such as a CLI tool or Git credential manager.
diff --git a/translations/zh-CN/content/developers/webhooks-and-events/webhook-events-and-payloads.md b/translations/zh-CN/content/developers/webhooks-and-events/webhook-events-and-payloads.md
index 84f7594162a4..eb4a105f3016 100644
--- a/translations/zh-CN/content/developers/webhooks-and-events/webhook-events-and-payloads.md
+++ b/translations/zh-CN/content/developers/webhooks-and-events/webhook-events-and-payloads.md
@@ -1288,7 +1288,7 @@ Web 挂钩事件是基于您注册的域的特异性而触发的。 例如,如
{{ webhookPayloadsForCurrentVersion.watch.started }}
-{% if currentVersion == "free-pro-team@latest" %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
### workflow_dispatch
This event occurs when someone triggers a workflow run on GitHub or sends a `POST` request to the "[Create a workflow dispatch event](/rest/reference/actions/#create-a-workflow-dispatch-event)" endpoint. 更多信息请参阅“[触发工作流程的事件](/actions/reference/events-that-trigger-workflows#workflow_dispatch)”。
@@ -1302,9 +1302,10 @@ This event occurs when someone triggers a workflow run on GitHub or sends a `POS
{{ webhookPayloadsForCurrentVersion.workflow_dispatch }}
{% endif %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" %}
### workflow_run
-When a {% data variables.product.prodname_actions %} workflow run is requested or completed. 更多信息请参阅“[触发工作流程的事件](/actions/reference/events-that-trigger-workflows#workflow_run)”。
+When a {% data variables.product.prodname_actions %} workflow run is requested or completed. For more information, see "[Events that trigger workflows](/actions/reference/events-that-trigger-workflows#workflow_run)."
#### 可用性
@@ -1322,3 +1323,4 @@ When a {% data variables.product.prodname_actions %} workflow run is requested o
#### Web 挂钩有效负载示例
{{ webhookPayloadsForCurrentVersion.workflow_run }}
+{% endif %}
diff --git a/translations/zh-CN/content/github/administering-a-repository/about-secret-scanning.md b/translations/zh-CN/content/github/administering-a-repository/about-secret-scanning.md
index 091d87fdc51e..ffc5d7f77949 100644
--- a/translations/zh-CN/content/github/administering-a-repository/about-secret-scanning.md
+++ b/translations/zh-CN/content/github/administering-a-repository/about-secret-scanning.md
@@ -18,6 +18,8 @@ versions:
### 关于公共仓库的 {% data variables.product.prodname_secret_scanning %}
+ {% data variables.product.prodname_secret_scanning_caps %} is automatically enabled on public repositories, where it scans code for secrets, to check for known secret formats. When a match of your secret format is found in a public repository, {% data variables.product.company_short %} doesn't publicly disclose the information as an alert, but instead sends a payload to an HTTP endpoint of your choice. For an overview of how secret scanning works on public repositories, see "[Secret scanning](/developers/overview/secret-scanning)."
+
当您推送到公共仓库时,{% data variables.product.product_name %} 会扫描提交的内容中是否有密码。 如果将私有仓库切换到公共仓库,{% data variables.product.product_name %} 会扫描整个仓库中的密码。
当 {% data variables.product.prodname_secret_scanning %} 检测一组凭据时,我们会通知发布密码的服务提供商。 服务提供商会验证该凭据,然后决定是否应撤销密钥、颁发新密钥或直接与您联系,具体取决于与您或服务提供商相关的风险。
@@ -65,6 +67,8 @@ versions:
{% data reusables.secret-scanning.beta %}
+If you're a repository administrator or an organization owner, you can enable {% data variables.product.prodname_secret_scanning %} for private repositories that are owned by organizations. You can enable {% data variables.product.prodname_secret_scanning %} for all your repositories, or for all new repositories within your organization. {% data variables.product.prodname_secret_scanning_caps %} is not available for user account-owned private repositories. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository)" and "[Managing security and analysis settings for your organization](/github/setting-up-and-managing-organizations-and-teams/managing-security-and-analysis-settings-for-your-organization)."
+
将提交推送到启用了 {% data variables.product.prodname_secret_scanning %} 的私有仓库时,{% data variables.product.product_name %} 会扫描提交的内容中是否有密码:
当 {% data variables.product.prodname_secret_scanning %} 在私有仓库中检测到密码时,{% data variables.product.prodname_dotcom %} 会发送警报。
@@ -73,6 +77,8 @@ versions:
- {% data variables.product.prodname_dotcom %} 在仓库中显示警报。 更多信息请参阅“[管理来自 {% data variables.product.prodname_secret_scanning %} 的警报](/github/administering-a-repository/managing-alerts-from-secret-scanning)”。
+Repository administrators and organization owners can grant users and team access to {% data variables.product.prodname_secret_scanning %} alerts. 更多信息请参阅“[管理仓库的安全和分析设置](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-security-alerts)”。
+
{% data variables.product.product_name %} 当前会扫描私有仓库,查找以下服务提供商发布的密码。
- Adafruit
diff --git a/translations/zh-CN/content/github/administering-a-repository/classifying-your-repository-with-topics.md b/translations/zh-CN/content/github/administering-a-repository/classifying-your-repository-with-topics.md
index 57b7fa99464e..4ed3cde0bcf7 100644
--- a/translations/zh-CN/content/github/administering-a-repository/classifying-your-repository-with-topics.md
+++ b/translations/zh-CN/content/github/administering-a-repository/classifying-your-repository-with-topics.md
@@ -22,7 +22,7 @@ versions:
仓库管理员可以添加他们喜欢的任何主题到仓库。 适用于对仓库分类的主题包括仓库的预期目的、主题领域、社区或语言。{% if currentVersion == "free-pro-team@latest" %}此外,{% data variables.product.product_name %} 也会分析公共仓库内容,生成建议的主题,仓库管理员可以接受或拒绝。 私有仓库内容不可分析,也不会收到主题建议。{% endif %}
-公共和私有仓库都可有主题,但在主题搜索结果中只能看到您可以访问的私有仓库。
+{% if currentVersion == "github-ae@latest" %}Internal {% else %}Public, internal, {% endif %}and private repositories can have topics, although you will only see private repositories that you have access to in topic search results.
您可以搜索与公共仓库关联的仓库。 更多信息请参阅“[搜索仓库](/articles/searching-for-repositories#search-by-topic)”。 您也可以搜索 {% data variables.product.product_name %} 中的主题列表。 更多信息请参阅“[搜索主题](/articles/searching-topics)”。
diff --git a/translations/zh-CN/content/github/administering-a-repository/customizing-your-repositorys-social-media-preview.md b/translations/zh-CN/content/github/administering-a-repository/customizing-your-repositorys-social-media-preview.md
index 8bf89a3c70db..48f764aef79b 100644
--- a/translations/zh-CN/content/github/administering-a-repository/customizing-your-repositorys-social-media-preview.md
+++ b/translations/zh-CN/content/github/administering-a-repository/customizing-your-repositorys-social-media-preview.md
@@ -11,7 +11,7 @@ versions:
在添加图像之前,请展开仓库链接以显示关于仓库和所有者头像的基本信息。 为仓库添加图像有助于在各种社交平台上识别您的项目。
-可以向私有仓库上传图像,但只能从公共仓库下载图像。
+{% if currentVersion != "github-ae@latest" %}You can upload an image to a private repository, but your image can only be shared from a public repository.{% endif %}
{% tip %}
提示:您的图像应为大小在 1 MB 以下的 PNG、JPG 或 GIF 文件。 为获取质量最佳的渲染,建议图像的像素保持在 640 x 320 像素。
diff --git a/translations/zh-CN/content/github/administering-a-repository/deleting-a-repository.md b/translations/zh-CN/content/github/administering-a-repository/deleting-a-repository.md
index f367d6d087fe..a921d075b747 100644
--- a/translations/zh-CN/content/github/administering-a-repository/deleting-a-repository.md
+++ b/translations/zh-CN/content/github/administering-a-repository/deleting-a-repository.md
@@ -13,17 +13,16 @@ versions:
{% data reusables.organizations.owners-and-admins-can %} 删除组织仓库。 如果已禁用 **Allow members to delete or transfer repositories for this organization(允许成员删除或转让此组织的仓库)**,仅组织所有者可删除组织仓库。 {% data reusables.organizations.new-repo-permissions-more-info %}
-{% if currentVersion == "free-pro-team@latest" %}
+{% if currentVersion != "github-ae@latest" %}Deleting a public repository will not delete any forks of the repository.{% endif %}
+
{% warning %}
-**警告**:删除仓库将**永久**删除发行版附件和团队权限。 此操作**必须**完成。
+**警告**:
-{% endwarning %}
-{% endif %}
+- Deleting a repository will **permanently** delete release attachments and team permissions. 此操作**必须**完成。
+- Deleting a private {% if currentVersion ver_gt "enterprise-server@2.19" or currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" %}or internal {% endif %}repository will delete all forks of the repository.
-请同时记住进行以下内容:
-- 删除私有仓库将删除其所有复刻。
-- 删除公共仓库不会删除其复刻。
+{% endwarning %}
{% if currentVersion == "free-pro-team@latest" %}
您可以在 90 天内恢复一些已删除的仓库。 更多信息请参阅“[恢复删除的仓库](/articles/restoring-a-deleted-repository)”。
diff --git a/translations/zh-CN/content/github/administering-a-repository/enabling-anonymous-git-read-access-for-a-repository.md b/translations/zh-CN/content/github/administering-a-repository/enabling-anonymous-git-read-access-for-a-repository.md
index 3b2b97f9f4b1..3e6d9f7b4b95 100644
--- a/translations/zh-CN/content/github/administering-a-repository/enabling-anonymous-git-read-access-for-a-repository.md
+++ b/translations/zh-CN/content/github/administering-a-repository/enabling-anonymous-git-read-access-for-a-repository.md
@@ -5,7 +5,6 @@ redirect_from:
- /articles/enabling-anonymous-git-read-access-for-a-repository
versions:
enterprise-server: '*'
- github-ae: '*'
---
在以下情况下,仓库管理员可以更改特定仓库的匿名 Git 读取权限设置:
diff --git a/translations/zh-CN/content/github/administering-a-repository/managing-repository-settings.md b/translations/zh-CN/content/github/administering-a-repository/managing-repository-settings.md
index 82edbb4f7bb6..818e46ede949 100644
--- a/translations/zh-CN/content/github/administering-a-repository/managing-repository-settings.md
+++ b/translations/zh-CN/content/github/administering-a-repository/managing-repository-settings.md
@@ -1,6 +1,6 @@
---
title: 管理仓库设置
-intro: '仓库管理员和组织所有者可以更改多项设置,包括仓库的名称和所有权,以及仓库的公有或私有可见性。 他们还可以删除仓库。'
+intro: 'Repository administrators and organization owners can change settings for a repository, like the name, ownership, and visibility, or delete the repository.'
mapTopic: true
redirect_from:
- /articles/managing-repository-settings
diff --git a/translations/zh-CN/content/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository.md b/translations/zh-CN/content/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository.md
index bd6e7acafbd3..f8c7b40976d2 100644
--- a/translations/zh-CN/content/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository.md
+++ b/translations/zh-CN/content/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository.md
@@ -22,28 +22,28 @@ versions:
{% data reusables.repositories.navigate-to-security-and-analysis %}
4. 在“Configure security and analysis features(配置安全性和分析功能)”下,单击功能右侧的 **Disable(禁用)**或 **Enable(启用)**。 
-### 授予对 {% data variables.product.prodname_dependabot_alerts %} 的访问
+### 授予对安全警报的访问权限
-为组织中的仓库启用 {% data variables.product.prodname_dependabot_alerts %} 后,组织所有者和仓库管理员默认可以查看警报。 您可以授予其他团队和人员访问仓库的警报。
+After you enable {% data variables.product.prodname_dependabot %} or {% data variables.product.prodname_secret_scanning %} alerts for a repository in an organization, organization owners and repository administrators can view the alerts by default. 您可以授予其他团队和人员访问仓库的警报。
{% note %}
-组织所有者和仓库管理员只能将查看 {% data variables.product.prodname_dependabot_alerts %} 的权限授予对仓库有写入权限的人员或团队。
+Organization owners and repository administrators can only grant access to view security alerts, such as {% data variables.product.prodname_dependabot %} and {% data variables.product.prodname_secret_scanning %} alerts, to people or teams who have write access to the repo.
{% endnote %}
{% data reusables.repositories.navigate-to-repo %}
{% data reusables.repositories.sidebar-settings %}
{% data reusables.repositories.navigate-to-security-and-analysis %}
-4. 在“Dependabot alerts(Dependabot 警报)”下,在搜索字段中开始键入您要查找的个人或团队的名称,然后单击匹配列表中的名称。 
-5. 单击 **Save changes(保存更改)**。 
+4. Under "Access to alerts", in the search field, start typing the name of the person or team you'd like to find, then click a name in the list of matches. 
+5. 单击 **Save changes(保存更改)**。 
-### 删除对 {% data variables.product.prodname_dependabot_alerts %} 的访问
+### 删除对安全警报的访问权限
{% data reusables.repositories.navigate-to-repo %}
{% data reusables.repositories.sidebar-settings %}
{% data reusables.repositories.navigate-to-security-and-analysis %}
-4. 在“Dependabot alerts(Dependabot 警报)”下,在要删除其访问权限的个人或团队的右侧,单击 {% octicon "x" aria-label="X symbol" %}。 
+4. Under "Access to alerts", to the right of the person or team whose access you'd like to remove, click {% octicon "x" aria-label="X symbol" %}. 
### 延伸阅读
diff --git a/translations/zh-CN/content/github/administering-a-repository/setting-repository-visibility.md b/translations/zh-CN/content/github/administering-a-repository/setting-repository-visibility.md
index 0948548fd032..529b7772c05c 100644
--- a/translations/zh-CN/content/github/administering-a-repository/setting-repository-visibility.md
+++ b/translations/zh-CN/content/github/administering-a-repository/setting-repository-visibility.md
@@ -19,17 +19,36 @@ versions:
我们建议在您更改仓库可见性之前审查以下注意事项。
#### 将仓库设为私有
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+* {% data variables.product.product_name %} 将会分离公共仓库的公共复刻并将其放入新的网络中。 Public forks are not made private.{% endif %}
+* If you change a repository's visibility from internal to private, {% data variables.product.prodname_dotcom %} will remove forks that belong to any user without access to the newly private repository. {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}The visibility of any forks will also change to private.{% elsif currentVersion == "github-ae@latest" %}If the internal repository has any forks, the visibility of the forks is already private.{% endif %} For more information, see "[What happens to forks when a repository is deleted or changes visibility?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility)"{% if currentVersion == "free-pro-team@latest" %}
+* If you're using {% data variables.product.prodname_free_user %} for user accounts or organizations, some features won't be available in the repository after you change the visibility to private. {% data reusables.gated-features.more-info %}{% endif %}
+* Any published {% data variables.product.prodname_pages %} site will be automatically unpublished.{% if currentVersion == "free-pro-team@latest" %} If you added a custom domain to the {% data variables.product.prodname_pages %} site, you should remove or update your DNS records before making the repository private, to avoid the risk of a domain takeover. For more information, see "[Managing a custom domain for your {% data variables.product.prodname_pages %} site](/articles/managing-a-custom-domain-for-your-github-pages-site)."{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+* {% data variables.product.prodname_dotcom %} 不再在 {% data variables.product.prodname_archive %} 中包含该仓库。 For more information, see "[About archiving content and data on {% data variables.product.prodname_dotcom %}](/github/creating-cloning-and-archiving-repositories/about-archiving-content-and-data-on-github#about-the-github-archive-program)."{% endif %}{% if enterpriseServerVersions contains currentVersion %}
+* Anonymous Git read access is no longer available. 更多信息请参阅“[为仓库启用匿名 Git 读取权限](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)”。{% endif %}
- * {% data variables.product.prodname_dotcom %} 将会分离公共仓库的公共复刻并将其放入新的网络中。 公共复刻无法设为私有。 {% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %}如果将仓库的可见性从内部更改为私有,{% data variables.product.prodname_dotcom %} 将删除属于无法访问新私有仓库的任何用户的复刻。{% endif %}更多信息请参阅“[删除仓库或更改其可见性时,复刻会发生什么变化?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility#changing-a-public-repository-to-a-private-repository)”
- {% if currentVersion == "free-pro-team@latest" %}* 如果对用户帐户或组织使用 {% data variables.product.prodname_free_user %},有些功能在您将可见性更改为私有后不可用于仓库。 {% data reusables.gated-features.more-info %}
- * 任何已发布的 {% data variables.product.prodname_pages %} 站点都将自动取消发布。 如果您将自定义域添加到 {% data variables.product.prodname_pages %} 站点,应在将仓库设为私有之前删除或更新 DNS 记录,以避免域接管的风险。 更多信息请参阅“[管理 {% data variables.product.prodname_pages %} 网站的自定义域](/articles/managing-a-custom-domain-for-your-github-pages-site)。
- * {% data variables.product.prodname_dotcom %} 不再在 {% data variables.product.prodname_archive %} 中包含该仓库。 更多信息请参阅“[关于在 {% data variables.product.prodname_dotcom %}](/github/creating-cloning-and-archiving-repositories/about-archiving-content-and-data-on-github#about-the-github-archive-program) 上存档内容和数据”。{% endif %}
- {% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}* 匿名 Git 读取权限不再可用。 更多信息请参阅“[为仓库启用匿名 Git 读取权限](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)”。{% endif %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %}
+
+#### 将仓库设为内部
+
+{% note %}
+
+**注:**{% data reusables.gated-features.internal-repos %}
+
+{% endnote %}
+
+* Any forks of the repository will remain in the repository network, and {% data variables.product.product_name %} maintains the relationship between the root repository and the fork. For more information, see "[What happens to forks when a repository is deleted or changes visibility?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility)"
+
+{% endif %}
+
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
#### 将仓库设为公共
- * {% data variables.product.prodname_dotcom %} 将会分离私有复刻并将它们变成独立的私有仓库。 更多信息请参阅“[删除仓库或更改其可见性时,复刻会发生什么变化?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility#changing-a-private-repository-to-a-public-repository)”
- * 如果您将私有仓库转换为公共仓库作为转向创建开源项目的组成部分, 请参阅[开源指南](http://opensource.guide)以获得有用的提示和指导。{% if currentVersion == "free-pro-team@latest" %}您还可以通过 [{% data variables.product.prodname_learning %}]({% data variables.product.prodname_learning_link %}) 参加有关管理开源项目的免费课程。 您的仓库设为公共后,您还可以查看仓库的社区资料以了解项目是否符合支持贡献者的最佳做法。 更多信息请参阅“[查看您的社区资料](/articles/viewing-your-community-profile)”。{% endif %}
+* {% data variables.product.product_name %} will detach private forks and turn them into a standalone private repository. For more information, see "[What happens to forks when a repository is deleted or changes visibility?](/articles/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility#changing-a-private-repository-to-a-public-repository)"{% if currentVersion == "free-pro-team@latest" %}
+* If you're converting your private repository to a public repository as part of a move toward creating an open source project, see the [Open Source Guides](http://opensource.guide) for helpful tips and guidelines. You can also take a free course on managing an open source project with [{% data variables.product.prodname_learning %}]({% data variables.product.prodname_learning_link %}). 您的仓库设为公共后,您还可以查看仓库的社区资料以了解项目是否符合支持贡献者的最佳做法。 更多信息请参阅“[查看您的社区资料](/articles/viewing-your-community-profile)”。{% endif %}
+
+{% endif %}
{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
diff --git a/translations/zh-CN/content/github/authenticating-to-github/about-anonymized-image-urls.md b/translations/zh-CN/content/github/authenticating-to-github/about-anonymized-image-urls.md
index d20403f6c238..5f862793ab8f 100644
--- a/translations/zh-CN/content/github/authenticating-to-github/about-anonymized-image-urls.md
+++ b/translations/zh-CN/content/github/authenticating-to-github/about-anonymized-image-urls.md
@@ -8,7 +8,7 @@ versions:
free-pro-team: '*'
---
-为托管您的图像,{% data variables.product.product_name %} 使用 [开源项目 Camo](https://github.com/atmos/camo)。 Camo 为每幅图像生成开头为 `https://camo.githubusercontent.com/` 的匿名 URL 代理,将会对其他用户隐藏您的浏览器详细信息和相关信息。
+为托管您的图像,{% data variables.product.product_name %} 使用 [开源项目 Camo](https://github.com/atmos/camo)。 Camo generates an anonymous URL proxy for each image which hides your browser details and related information from other users. The URL starts `https://.githubusercontent.com/`, with different subdomains depending on how you uploaded the image.
直接或间接收到您的匿名化图像 URL 的任何人都可查看您的图像。 为对敏感图像保密,将它们限于私人网络或需要身份验证的服务器,而不使用 Camo。
diff --git a/translations/zh-CN/content/github/authenticating-to-github/adding-a-new-ssh-key-to-your-github-account.md b/translations/zh-CN/content/github/authenticating-to-github/adding-a-new-ssh-key-to-your-github-account.md
index b4e6c6aaef0c..ee48dd78e591 100644
--- a/translations/zh-CN/content/github/authenticating-to-github/adding-a-new-ssh-key-to-your-github-account.md
+++ b/translations/zh-CN/content/github/authenticating-to-github/adding-a-new-ssh-key-to-your-github-account.md
@@ -24,8 +24,8 @@ versions:
如果您的 SSH 密钥文件与示例代码不同,请修改文件名以匹配您当前的设置。 在复制密钥时,请勿添加任何新行或空格。
```shell
- $ pbcopy < ~/.ssh/id_rsa.pub
- # Copies the contents of the id_rsa.pub file to your clipboard
+ $ pbcopy < ~/.ssh/id_ed25519.pub
+ # Copies the contents of the id_ed25519.pub file to your clipboard
```
{% tip %}
@@ -51,8 +51,8 @@ versions:
如果您的 SSH 密钥文件与示例代码不同,请修改文件名以匹配您当前的设置。 在复制密钥时,请勿添加任何新行或空格。
```shell
- $ clip < ~/.ssh/id_rsa.pub
- # Copies the contents of the id_rsa.pub file to your clipboard
+ $ clip < ~/.ssh/id_ed25519.pub
+ # Copies the contents of the id_ed25519.pub file to your clipboard
```
{% tip %}
@@ -81,8 +81,8 @@ versions:
$ sudo apt-get install xclip
# Downloads and installs xclip. If you don't have `apt-get`, you might need to use another installer (like `yum`)
- $ xclip -selection clipboard < ~/.ssh/id_rsa.pub
- # Copies the contents of the id_rsa.pub file to your clipboard
+ $ xclip -selection clipboard < ~/.ssh/id_ed25519.pub
+ # Copies the contents of the id_ed25519.pub file to your clipboard
```
{% tip %}
diff --git a/translations/zh-CN/content/github/authenticating-to-github/generating-a-new-ssh-key-and-adding-it-to-the-ssh-agent.md b/translations/zh-CN/content/github/authenticating-to-github/generating-a-new-ssh-key-and-adding-it-to-the-ssh-agent.md
index 279fb5db448c..d29629f2d2a5 100644
--- a/translations/zh-CN/content/github/authenticating-to-github/generating-a-new-ssh-key-and-adding-it-to-the-ssh-agent.md
+++ b/translations/zh-CN/content/github/authenticating-to-github/generating-a-new-ssh-key-and-adding-it-to-the-ssh-agent.md
@@ -98,13 +98,19 @@ versions:
IdentityFile ~/.ssh/id_ed25519
```
+ {% note %}
+
+ **Note:** If you chose not to add a passphrase to your key, you should omit the `UseKeychain` line.
+
+ {% endnote %}
+
3. 将 SSH 私钥添加到 ssh-agent 并将密码存储在密钥链中。 {% data reusables.ssh.add-ssh-key-to-ssh-agent %}
```shell
$ ssh-add -K ~/.ssh/id_ed25519
```
{% note %}
- **注:**`-K` 选项位于 Apple 的 `ssh-add` 标准版本中,当您将 ssh 密钥添加到 ssh-agent 时,它会将密码存储在您的密钥链中。
+ **注:**`-K` 选项位于 Apple 的 `ssh-add` 标准版本中,当您将 ssh 密钥添加到 ssh-agent 时,它会将密码存储在您的密钥链中。 If you chose not to add a passphrase to your key, run the command without the `-K` option.
如果您没有安装 Apple 的标准版本,可能会收到错误消息。 有关解决此错误的详细信息,请参阅“[错误:ssh-add:非法选项 -- K](/articles/error-ssh-add-illegal-option-k)”。
diff --git a/translations/zh-CN/content/github/authenticating-to-github/reviewing-your-security-log.md b/translations/zh-CN/content/github/authenticating-to-github/reviewing-your-security-log.md
index e178adaafdbe..bbf9ce37bc8b 100644
--- a/translations/zh-CN/content/github/authenticating-to-github/reviewing-your-security-log.md
+++ b/translations/zh-CN/content/github/authenticating-to-github/reviewing-your-security-log.md
@@ -1,6 +1,7 @@
---
title: 审查您的安全日志
intro: 您可以查看用户帐户的安全日志,以更好地了解您执行的操作以及其他人执行的与您有关的操作。
+miniTocMaxHeadingLevel: 4
redirect_from:
- /articles/reviewing-your-security-log
versions:
@@ -30,216 +31,222 @@ versions:
#### 基于执行的操作搜索
{% else %}
### 了解安全日志中的事件
+{% endif %}
+
+The events listed in your security log are triggered by your actions. Actions are grouped into the following categories:
+
+| 类别名称 | 描述 |
+| -------------------------------------------------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |{% if currentVersion == "free-pro-team@latest" %}
+| [`account_recovery_token`](#account_recovery_token-category-actions) | 包含与[添加恢复令牌](/articles/configuring-two-factor-authentication-recovery-methods)相关的所有活动。 |
+| [`计费,帐单`](#billing-category-actions) | 包含与帐单信息相关的所有活动。 |
+| [`marketplace_agreement_signature`](#marketplace_agreement_signature-category-actions) | 包含与签署 {% data variables.product.prodname_marketplace %} 开发者协议相关的所有活动。 |
+| [`marketplace_listing`](#marketplace_listing-category-actions) | 包含与 {% data variables.product.prodname_marketplace %} 中列出的应用程序相关的所有活动。{% endif %}
+| [`oauth_access`](#oauth_access-category-actions) | 包含与您已连接的 [{% data variables.product.prodname_oauth_app %}](/articles/authorizing-oauth-apps) 相关的所有活动。{% if currentVersion == "free-pro-team@latest" %}
+| [`payment_method`](#payment_method-category-actions) | 包含与 {% data variables.product.prodname_dotcom %} 订阅支付相关的所有活动。{% endif %}
+| [`profile_picture`](#profile_picture-category-actions) | 包含与头像相关的所有活动。 |
+| [`project`](#project-category-actions) | 包含与项目板相关的所有活动。 |
+| [`public_key`](#public_key-category-actions) | 包含与[公共 SSH 密钥](/articles/adding-a-new-ssh-key-to-your-github-account)相关的所有活动。 |
+| [`repo`](#repo-category-actions) | 包含与您拥有的仓库相关的所有活动。{% if currentVersion == "free-pro-team@latest" %}
+| [`sponsors`](#sponsors-category-actions) | 包含与 {% data variables.product.prodname_sponsors %}和赞助者按钮相关的所有事件(请参阅“[关于 {% data variables.product.prodname_sponsors %}](/articles/about-github-sponsors)”和“[在仓库中显示赞助者按钮](/articles/displaying-a-sponsor-button-in-your-repository)”){% endif %}{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
+| [`团队`](#team-category-actions) | 包含与您所在团队相关的所有活动。{% endif %}{% if currentVersion != "github-ae@latest" %}
+| [`two_factor_authentication`](#two_factor_authentication-category-actions) | 包含与[双重身份验证](/articles/securing-your-account-with-two-factor-authentication-2fa)相关的所有活动。{% endif %}
+| [`用户`](#user-category-actions) | 包含与您的帐户相关的所有活动。 |
+
+{% if currentVersion == "free-pro-team@latest" %}
+
+### 导出安全日志
+
+{% data reusables.audit_log.export-log %}
+{% data reusables.audit_log.exported-log-keys-and-values %}
-安全日志中列出的操作分为以下类别: |{% endif %}
-| 类别名称 | 描述 |
-| ------------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |{% if currentVersion == "free-pro-team@latest" %}
-| `account_recovery_token` | 包含与[添加恢复令牌](/articles/configuring-two-factor-authentication-recovery-methods)相关的所有活动。 |
-| `计费,帐单` | 包含与帐单信息相关的所有活动。 |
-| `marketplace_agreement_signature` | 包含与签署 {% data variables.product.prodname_marketplace %} 开发者协议相关的所有活动。 |
-| `marketplace_listing` | 包含与 {% data variables.product.prodname_marketplace %} 中列出的应用程序相关的所有活动。{% endif %}
-| `oauth_access` | 包含与您已连接的 [{% data variables.product.prodname_oauth_app %}](/articles/authorizing-oauth-apps) 相关的所有活动。{% if currentVersion == "free-pro-team@latest" %}
-| `payment_method` | 包含与 {% data variables.product.prodname_dotcom %} 订阅支付相关的所有活动。{% endif %}
-| `profile_picture` | 包含与头像相关的所有活动。 |
-| `project` | 包含与项目板相关的所有活动。 |
-| `public_key` | 包含与[公共 SSH 密钥](/articles/adding-a-new-ssh-key-to-your-github-account)相关的所有活动。 |
-| `repo` | 包含与您拥有的仓库相关的所有活动。{% if currentVersion == "free-pro-team@latest" %}
-| `sponsors` | 包含与 {% data variables.product.prodname_sponsors %}和赞助者按钮相关的所有事件(请参阅“[关于 {% data variables.product.prodname_sponsors %}](/articles/about-github-sponsors)”和“[在仓库中显示赞助者按钮](/articles/displaying-a-sponsor-button-in-your-repository)”){% endif %}{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-| `团队` | 包含与您所在团队相关的所有活动。{% endif %}{% if currentVersion != "github-ae@latest" %}
-| `two_factor_authentication` | 包含与[双重身份验证](/articles/securing-your-account-with-two-factor-authentication-2fa)相关的所有活动。{% endif %}
-| `用户` | 包含与您的帐户相关的所有活动。 |
-
-下面列出了这些类别中各事件的说明。
+{% endif %}
+
+### Security log actions
+
+An overview of some of the most common actions that are recorded as events in the security log.
{% if currentVersion == "free-pro-team@latest" %}
-#### `account_recovery_token` 类别
+#### `account_recovery_token` category actions
-| 操作 | 描述 |
-| ------------- | ----------------------------------------------------------------------------------------- |
-| confirm | 当您成功[使用恢复提供程序存储新令牌](/articles/configuring-two-factor-authentication-recovery-methods)时触发。 |
-| recover | 当您成功[取回帐户恢复令牌](/articles/recovering-your-account-if-you-lose-your-2fa-credentials)时触发。 |
-| recover_error | 当 {% data variables.product.prodname_dotcom %} 无法验证所使用的令牌时触发。 |
+| 操作 | 描述 |
+| --------------- | ----------------------------------------------------------------------------------------- |
+| `confirm` | 当您成功[使用恢复提供程序存储新令牌](/articles/configuring-two-factor-authentication-recovery-methods)时触发。 |
+| `recover` | 当您成功[取回帐户恢复令牌](/articles/recovering-your-account-if-you-lose-your-2fa-credentials)时触发。 |
+| `recover_error` | 当 {% data variables.product.prodname_dotcom %} 无法验证所使用的令牌时触发。 |
-#### `billing` 类别
+#### `billing` category actions
| 操作 | 描述 |
| --------------------- | ----------------------------------------------------------------------------------------------------------- |
-| change_billing_type | 当您[更改 {% data variables.product.prodname_dotcom %} 的支付方式](/articles/adding-or-editing-a-payment-method)时触发。 |
-| change_email | 当您[更改您的电子邮件地址](/articles/changing-your-primary-email-address)时触发。 |
+| `change_billing_type` | 当您[更改 {% data variables.product.prodname_dotcom %} 的支付方式](/articles/adding-or-editing-a-payment-method)时触发。 |
+| `change_email` | 当您[更改您的电子邮件地址](/articles/changing-your-primary-email-address)时触发。 |
-#### `marketplace_agreement_signature` 类别
+#### `marketplace_agreement_signature` category actions
-| 操作 | 描述 |
-| ------ | --------------------------------------------------------------- |
-| create | 在签署 {% data variables.product.prodname_marketplace %} 开发者协议时触发。 |
+| 操作 | 描述 |
+| -------- | --------------------------------------------------------------- |
+| `create` | 在签署 {% data variables.product.prodname_marketplace %} 开发者协议时触发。 |
-#### `marketplace_listing` 类别
+#### `marketplace_listing` category actions
-| 操作 | 描述 |
-| ------- | -------------------------------------------------------------------- |
-| 批准 | 当您的列表被批准包含在 {% data variables.product.prodname_marketplace %} 中时触发。 |
-| create | 当您在 {% data variables.product.prodname_marketplace %} 中为应用程序创建列表时触发。 |
-| delist | 当您的列表从 {% data variables.product.prodname_marketplace %} 中被删除时触发。 |
-| redraft | 将您的列表被返回到草稿状态时触发。 |
-| reject | 当您的列表被拒绝包含在 {% data variables.product.prodname_marketplace %} 中时触发。 |
+| 操作 | 描述 |
+| --------- | -------------------------------------------------------------------- |
+| `批准` | 当您的列表被批准包含在 {% data variables.product.prodname_marketplace %} 中时触发。 |
+| `create` | 当您在 {% data variables.product.prodname_marketplace %} 中为应用程序创建列表时触发。 |
+| `delist` | 当您的列表从 {% data variables.product.prodname_marketplace %} 中被删除时触发。 |
+| `redraft` | 将您的列表被返回到草稿状态时触发。 |
+| `reject` | 当您的列表被拒绝包含在 {% data variables.product.prodname_marketplace %} 中时触发。 |
{% endif %}
-#### `oauth_access` 类别
+#### `oauth_access` category actions
-| 操作 | 描述 |
-| ------- | ------------------------------------------------------------------------------------------------------------------------ |
-| create | 当您[授予 {% data variables.product.prodname_oauth_app %} 访问权限](/articles/authorizing-oauth-apps)时触发。 |
-| destroy | 当您[撤销 {% data variables.product.prodname_oauth_app %} 对您帐户的访问权限](/articles/reviewing-your-authorized-integrations)时触发。 |
+| 操作 | 描述 |
+| --------- | ------------------------------------------------------------------------------------------------------------------------ |
+| `create` | 当您[授予 {% data variables.product.prodname_oauth_app %} 访问权限](/articles/authorizing-oauth-apps)时触发。 |
+| `destroy` | 当您[撤销 {% data variables.product.prodname_oauth_app %} 对您帐户的访问权限](/articles/reviewing-your-authorized-integrations)时触发。 |
{% if currentVersion == "free-pro-team@latest" %}
-#### `payment_method` 类别
+#### `payment_method` category actions
-| 操作 | 描述 |
-| ------ | ------------------------------------------------------ |
-| clear | 当存档的[付款方式](/articles/removing-a-payment-method)被删除时触发。 |
-| create | 在添加新的付款方式(例如新的信用卡或 PayPal 帐户)时触发。 |
-| update | 当现有付款方式被更新时触发。 |
+| 操作 | 描述 |
+| -------- | ------------------------------------------------------ |
+| `clear` | 当存档的[付款方式](/articles/removing-a-payment-method)被删除时触发。 |
+| `create` | 在添加新的付款方式(例如新的信用卡或 PayPal 帐户)时触发。 |
+| `update` | 当现有付款方式被更新时触发。 |
{% endif %}
-#### `profile_picture` 类别
+#### `profile_picture` category actions
-| 操作 | 描述 |
-| ------ | ------------------------------------------------------------ |
-| update | 当您[设置或更新个人资料图片](/articles/setting-your-profile-picture/)时触发。 |
+| 操作 | 描述 |
+| -------- | ------------------------------------------------------------ |
+| `update` | 当您[设置或更新个人资料图片](/articles/setting-your-profile-picture/)时触发。 |
-#### `project` 类别
+#### `project` category actions
| 操作 | 描述 |
| ------------------------ | --------------------------------- |
+| `access` | 当项目板的可见性被更改时触发。 |
| `create` | 在创建项目板时触发。 |
| `rename` | 当项目板被重命名时触发。 |
| `update` | 当项目板被更新时触发。 |
| `delete` | 在删除项目板时触发。 |
| `link` | 当仓库被链接到项目板时触发。 |
| `unlink` | 当仓库从项目板解除链接时触发。 |
-| `project.access` | 当项目板的可见性被更改时触发。 |
| `update_user_permission` | 在项目板中添加或删除外部协作者时,或者他们的权限级别被更改时触发。 |
-#### `public_key` 类别
+#### `public_key` category actions
-| 操作 | 描述 |
-| ------ | ------------------------------------------------------------------------------------------------------------------------- |
-| create | 当您[为 {% data variables.product.product_name %} 帐户添加新公共 SSH 密钥](/articles/adding-a-new-ssh-key-to-your-github-account)时触发。 |
-| delete | 当您[删除 {% data variables.product.product_name %} 帐户的公共 SSH 密钥](/articles/reviewing-your-ssh-keys)时触发。 |
+| 操作 | 描述 |
+| -------- | ------------------------------------------------------------------------------------------------------------------------- |
+| `create` | 当您[为 {% data variables.product.product_name %} 帐户添加新公共 SSH 密钥](/articles/adding-a-new-ssh-key-to-your-github-account)时触发。 |
+| `delete` | 当您[删除 {% data variables.product.product_name %} 帐户的公共 SSH 密钥](/articles/reviewing-your-ssh-keys)时触发。 |
-#### `repo` 类别
+#### `repo` category actions
| 操作 | 描述 |
| ------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| access | 当您拥有的仓库[从“私有”切换到“公共”](/articles/making-a-private-repository-public)(反之亦然)时触发。 |
-| add_member | 当 {% data variables.product.product_name %} 用户 {% if currentVersion == "free-pro-team@latest" %}[被邀请协作使用](/articles/inviting-collaborators-to-a-personal-repository){% else %}[被授权协作使用](/articles/inviting-collaborators-to-a-personal-repository){% endif %}仓库时触发。 |
-| add_topic | 当仓库所有者向仓库[添加主题](/articles/classifying-your-repository-with-topics)时触发。 |
-| archived | 在仓库所有者[存档仓库](/articles/about-archiving-repositories)时触发。{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-| config.disable_anonymous_git_access | 当公共仓库中[禁用匿名 Git 读取权限](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)时触发。 |
-| config.enable_anonymous_git_access | 当公共仓库中[启用匿名 Git 读取权限](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)时触发。 |
-| config.lock_anonymous_git_access | 当仓库的[匿名 Git 读取权限设置被锁定](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)时触发。 |
-| config.unlock_anonymous_git_access | 当仓库的[匿名 Git 读取权限设置被解锁](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)时触发。{% endif %}
-| create | 在[创建新仓库](/articles/creating-a-new-repository)时触发。 |
-| destroy | 当[仓库被删除](/articles/deleting-a-repository)时触发。{% if currentVersion == "free-pro-team@latest" %}
-| 禁用 | 当仓库被禁用(例如,因[资金不足](/articles/unlocking-a-locked-account))时触发。{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-| 启用 | 在重新启用仓库时触发。{% endif %}
-| remove_member | 从[仓库中删除 {% data variables.product.product_name %} 用户的协作者身份](/articles/removing-a-collaborator-from-a-personal-repository)时触发。 |
-| remove_topic | 当仓库所有者从仓库中删除主题时触发。 |
-| rename | 当[仓库被重命名](/articles/renaming-a-repository)时触发。 |
-| 转让 | 当[仓库被转让](/articles/how-to-transfer-a-repository)时触发。 |
-| transfer_start | 在仓库转让即将发生时触发。 |
-| unarchived | 当仓库所有者取消存档仓库时触发。 |
+| `access` | 当您拥有的仓库[从“私有”切换到“公共”](/articles/making-a-private-repository-public)(反之亦然)时触发。 |
+| `add_member` | 当 {% data variables.product.product_name %} 用户 {% if currentVersion == "free-pro-team@latest" %}[被邀请协作使用](/articles/inviting-collaborators-to-a-personal-repository){% else %}[被授权协作使用](/articles/inviting-collaborators-to-a-personal-repository){% endif %}仓库时触发。 |
+| `add_topic` | 当仓库所有者向仓库[添加主题](/articles/classifying-your-repository-with-topics)时触发。 |
+| `archived` | 当仓库所有者[存档仓库](/articles/about-archiving-repositories)时触发。{% if enterpriseServerVersions contains currentVersion %}
+| `config.disable_anonymous_git_access` | 当公共仓库中[禁用匿名 Git 读取权限](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)时触发。 |
+| `config.enable_anonymous_git_access` | 当公共仓库中[启用匿名 Git 读取权限](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository)时触发。 |
+| `config.lock_anonymous_git_access` | 当仓库的[匿名 Git 读取权限设置被锁定](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)时触发。 |
+| `config.unlock_anonymous_git_access` | 当仓库的[匿名 Git 读取权限设置被解锁](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access)时触发。{% endif %}
+| `create` | 在[创建新仓库](/articles/creating-a-new-repository)时触发。 |
+| `destroy` | 当[仓库被删除](/articles/deleting-a-repository)时触发。{% if currentVersion == "free-pro-team@latest" %}
+| `禁用` | 当仓库被禁用(例如,因[资金不足](/articles/unlocking-a-locked-account))时触发。{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| `启用` | 在重新启用仓库时触发。{% endif %}
+| `remove_member` | 从[仓库中删除 {% data variables.product.product_name %} 用户的协作者身份](/articles/removing-a-collaborator-from-a-personal-repository)时触发。 |
+| `remove_topic` | 当仓库所有者从仓库中删除主题时触发。 |
+| `rename` | 当[仓库被重命名](/articles/renaming-a-repository)时触发。 |
+| `转让` | 当[仓库被转让](/articles/how-to-transfer-a-repository)时触发。 |
+| `transfer_start` | 在仓库转让即将发生时触发。 |
+| `unarchived` | 当仓库所有者取消存档仓库时触发。 |
{% if currentVersion == "free-pro-team@latest" %}
-#### `sponsors` 类别
-
-| 操作 | 描述 |
-| ----------------------------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| repo_funding_link_button_toggle | 在仓库中启用或禁用赞助按钮时触发(请参阅“[在仓库中显示赞助按钮](/articles/displaying-a-sponsor-button-in-your-repository)”) |
-| repo_funding_links_file_action | 更改仓库中的 FUNDING 文件时触发(请参阅“[在仓库中显示赞助按钮](/articles/displaying-a-sponsor-button-in-your-repository)”) |
-| sponsor_sponsorship_cancel | 当您取消赞助时触发(请参阅“[降级赞助](/articles/downgrading-a-sponsorship)”) |
-| sponsor_sponsorship_create | 当您赞助开发者时触发(请参阅“[赞助开源开发者](/github/supporting-the-open-source-community-with-github-sponsors/sponsoring-an-open-source-contributor#sponsoring-a-developer)”) |
-| sponsor_sponsorship_preference_change | 当您更改是否接收被赞助开发者的电子邮件更新时触发(请参阅“[管理赞助](/articles/managing-your-sponsorship)”) |
-| sponsor_sponsorship_tier_change | 当您升级或降级赞助时触发(请参阅“[升级赞助](/articles/upgrading-a-sponsorship)”和“[降级赞助](/articles/downgrading-a-sponsorship)”) |
-| sponsored_developer_approve | 当您的 {% data variables.product.prodname_sponsors %} 帐户被批准时触发(请参阅“[为您的用户帐户设置 {% data variables.product.prodname_sponsors %}](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)”) |
-| sponsored_developer_create | 当您的 {% data variables.product.prodname_sponsors %} 帐户创建时触发(请参阅“[为您的用户帐户设置 {% data variables.product.prodname_sponsors %}](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)”) |
-| sponsored_developer_profile_update | 在编辑您的被赞助开发者个人资料时触发(请参阅“[编辑 {% data variables.product.prodname_sponsors %} 的个人资料详细信息](/github/supporting-the-open-source-community-with-github-sponsors/editing-your-profile-details-for-github-sponsors)”) |
-| sponsored_developer_request_approval | 提交您对 {% data variables.product.prodname_sponsors %} 的申请以供审批时触发(请参阅“[为您的用户帐户设置 {% data variables.product.prodname_sponsors %}](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)”) |
-| sponsored_developer_tier_description_update | 当您更改赞助等级的说明时触发(请参阅“[更改赞助等级](/articles/changing-your-sponsorship-tiers)”) |
-| sponsored_developer_update_newsletter_send | 当您向赞助者发送电子邮件更新时触发(请参阅“[联系赞助者](/articles/contacting-your-sponsors)”) |
-| waitlist_invite_sponsored_developer | 当您从等候名单被邀请加入 {% data variables.product.prodname_sponsors %} 时触发(请参阅“[为您的用户帐户设置 {% data variables.product.prodname_sponsors %}](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)”) |
-| waitlist_join | 当您加入成为被赞助开发者的等候名单时触发(请参阅“[为您的用户帐户设置 {% data variables.product.prodname_sponsors %}](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)”) |
+#### `sponsors` category actions
+
+| 操作 | 描述 |
+| --------------------------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `repo_funding_link_button_toggle` | 在仓库中启用或禁用赞助按钮时触发(请参阅“[在仓库中显示赞助按钮](/articles/displaying-a-sponsor-button-in-your-repository)”) |
+| `repo_funding_links_file_action` | 更改仓库中的 FUNDING 文件时触发(请参阅“[在仓库中显示赞助按钮](/articles/displaying-a-sponsor-button-in-your-repository)”) |
+| `sponsor_sponsorship_cancel` | 当您取消赞助时触发(请参阅“[降级赞助](/articles/downgrading-a-sponsorship)”) |
+| `sponsor_sponsorship_create` | 当您赞助开发者时触发(请参阅“[赞助开源开发者](/github/supporting-the-open-source-community-with-github-sponsors/sponsoring-an-open-source-contributor#sponsoring-a-developer)”) |
+| `sponsor_sponsorship_preference_change` | 当您更改是否接收被赞助开发者的电子邮件更新时触发(请参阅“[管理赞助](/articles/managing-your-sponsorship)”) |
+| `sponsor_sponsorship_tier_change` | 当您升级或降级赞助时触发(请参阅“[升级赞助](/articles/upgrading-a-sponsorship)”和“[降级赞助](/articles/downgrading-a-sponsorship)”) |
+| `sponsored_developer_approve` | 当您的 {% data variables.product.prodname_sponsors %} 帐户被批准时触发(请参阅“[为您的用户帐户设置 {% data variables.product.prodname_sponsors %}](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)”) |
+| `sponsored_developer_create` | 当您的 {% data variables.product.prodname_sponsors %} 帐户创建时触发(请参阅“[为您的用户帐户设置 {% data variables.product.prodname_sponsors %}](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)”) |
+| `sponsored_developer_profile_update` | 在编辑您的被赞助开发者个人资料时触发(请参阅“[编辑 {% data variables.product.prodname_sponsors %} 的个人资料详细信息](/github/supporting-the-open-source-community-with-github-sponsors/editing-your-profile-details-for-github-sponsors)”) |
+| `sponsored_developer_request_approval` | 提交您对 {% data variables.product.prodname_sponsors %} 的申请以供审批时触发(请参阅“[为您的用户帐户设置 {% data variables.product.prodname_sponsors %}](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)”) |
+| `sponsored_developer_tier_description_update` | 当您更改赞助等级的说明时触发(请参阅“[更改赞助等级](/articles/changing-your-sponsorship-tiers)”) |
+| `sponsored_developer_update_newsletter_send` | 当您向赞助者发送电子邮件更新时触发(请参阅“[联系赞助者](/articles/contacting-your-sponsors)”) |
+| `waitlist_invite_sponsored_developer` | 当您从等候名单被邀请加入 {% data variables.product.prodname_sponsors %} 时触发(请参阅“[为您的用户帐户设置 {% data variables.product.prodname_sponsors %}](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)”) |
+| `waitlist_join` | 当您加入成为被赞助开发者的等候名单时触发(请参阅“[为您的用户帐户设置 {% data variables.product.prodname_sponsors %}](/github/supporting-the-open-source-community-with-github-sponsors/setting-up-github-sponsors-for-your-user-account)”) |
{% endif %}
{% if currentVersion == "free-pro-team@latest" %}
-#### `successor_invitation` 类别
-
-| 操作 | 描述 |
-| ------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| accept | 当您接受继承邀请时触发(请参阅“[保持用户帐户仓库的所有权连续性](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)”) |
-| cancel | 当您取消继承邀请时触发(请参阅“[保持用户帐户仓库的所有权连续性](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)”) |
-| create | 当您创建继承邀请时触发(请参阅“[保持用户帐户仓库的所有权连续性](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)”) |
-| decline | 当您拒绝继承邀请时触发(请参阅“[保持用户帐户仓库的所有权连续性](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)”) |
-| revoke | 当您撤销继承邀请时触发(请参阅“[保持用户帐户仓库的所有权连续性](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)”) |
+#### `successor_invitation` category actions
+
+| 操作 | 描述 |
+| --------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `accept` | 当您接受继承邀请时触发(请参阅“[保持用户帐户仓库的所有权连续性](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)”) |
+| `cancel` | 当您取消继承邀请时触发(请参阅“[保持用户帐户仓库的所有权连续性](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)”) |
+| `create` | 当您创建继承邀请时触发(请参阅“[保持用户帐户仓库的所有权连续性](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)”) |
+| `decline` | 当您拒绝继承邀请时触发(请参阅“[保持用户帐户仓库的所有权连续性](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)”) |
+| `revoke` | 当您撤销继承邀请时触发(请参阅“[保持用户帐户仓库的所有权连续性](/github/setting-up-and-managing-your-github-user-account/maintaining-ownership-continuity-of-your-user-accounts-repositories)”) |
{% endif %}
{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
-#### `team` 类别
+#### `team` category actions
-| 操作 | 描述 |
-| ----------------- | ------------------------------------------------------------------------ |
-| add_member | 当您所属组织的成员[将您添加到团队](/articles/adding-organization-members-to-a-team)时触发。 |
-| add_repository | 当您所属团队被授予控制仓库的权限时触发。 |
-| create | 当您所属组织中创建了新团队时触发。 |
-| destroy | 当您所属团队从组织中被删除时触发。 |
-| remove_member | [从您所属团队中删除组织成员](/articles/removing-organization-members-from-a-team)时触发。 |
-| remove_repository | 当仓库不再受团队控制时触发。 |
+| 操作 | 描述 |
+| ------------------- | ------------------------------------------------------------------------ |
+| `add_member` | 当您所属组织的成员[将您添加到团队](/articles/adding-organization-members-to-a-team)时触发。 |
+| `add_repository` | 当您所属团队被授予控制仓库的权限时触发。 |
+| `create` | 当您所属组织中创建了新团队时触发。 |
+| `destroy` | 当您所属团队从组织中被删除时触发。 |
+| `remove_member` | [从您所属团队中删除组织成员](/articles/removing-organization-members-from-a-team)时触发。 |
+| `remove_repository` | 当仓库不再受团队控制时触发。 |
{% endif %}
{% if currentVersion != "github-ae@latest" %}
-#### `two_factor_authentication` 类别
+#### `two_factor_authentication` category actions
-| 操作 | 描述 |
-| -------- | ----------------------------------------------------------------------------------- |
-| enabled | 在启用[双重身份验证](/articles/securing-your-account-with-two-factor-authentication-2fa)时触发。 |
-| disabled | 在禁用双重身份验证时触发。 |
+| 操作 | 描述 |
+| ---------- | ----------------------------------------------------------------------------------- |
+| `enabled` | 在启用[双重身份验证](/articles/securing-your-account-with-two-factor-authentication-2fa)时触发。 |
+| `disabled` | 在禁用双重身份验证时触发。 |
{% endif %}
-#### `user` 类别
-
-| 操作 | 描述 |
-| ---------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------- |
-| add_email | 在您{% if currentVersion != "github-ae@latest" %}[新增电子邮件地址](/articles/changing-your-primary-email-address){% else %}新增电子邮件地址{% endif %}时触发。 |
-| create | 当您创建新用户帐户时触发。 |
-| remove_email | 当您删除电子邮件地址时触发。 |
-| rename | 在重命名帐户时触发。{% if currentVersion != "github-ae@latest" %}
-| change_password | 当您更改密码时触发。 |
-| forgot_password | 在您要求[重置密码](/articles/how-can-i-reset-my-password)时触发。{% endif %}
-| login | 当您登录 {% data variables.product.product_location %} 时触发。 |
-| failed_login | 在无法成功登录时触发。{% if currentVersion != "github-ae@latest" %}
-| two_factor_requested | 当 {% data variables.product.product_name %} 要求您提供[双重身份验证代码](/articles/accessing-github-using-two-factor-authentication)时触发。{% endif %}
-| show_private_contributions_count | 当您[在个人资料中公开私有贡献](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)时触发。 |
-| hide_private_contributions_count | 当您[在个人资料中隐藏私有贡献](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)时触发。{% if currentVersion == "free-pro-team@latest" %}
-| report_content | 当您[举报议题或拉取请求,或者举报对议题、拉取请求或提交的评论](/articles/reporting-abuse-or-spam)时触发。{% endif %}
-
-#### `user_status` 类别
-
-| 操作 | 描述 |
-| ------- | -------------------------------------------------------------------------------------------- |
-| update | 当您在个人资料中设置或更改状态时触发。 更多信息请参阅“[设置状态](/articles/personalizing-your-profile/#setting-a-status)”。 |
-| destroy | 当您在个人资料中清除状态时触发。 |
+#### `user` category actions
-{% if currentVersion == "free-pro-team@latest" %}
+| 操作 | 描述 |
+| ---------------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `add_email` | 在您{% if currentVersion != "github-ae@latest" %}[新增电子邮件地址](/articles/changing-your-primary-email-address){% else %}新增电子邮件地址{% endif %}时触发。 |
+| `create` | Triggered when you create a new user account.{% if currentVersion != "github-ae@latest" %}
+| `change_password` | 当您更改密码时触发。 |
+| `forgot_password` | 在您要求[重置密码](/articles/how-can-i-reset-my-password)时触发。{% endif %}
+| `hide_private_contributions_count` | 当您[在个人资料中隐藏私有贡献](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)时触发。 |
+| `login` | 当您登录 {% data variables.product.product_location %} 时触发。 |
+| `failed_login` | 当您未能成功登录时触发。 |
+| `remove_email` | 当您删除电子邮件地址时触发。 |
+| `rename` | Triggered when you rename your account.{% if currentVersion == "free-pro-team@latest" %}
+| `report_content` | 当您[举报议题或拉取请求,或者举报对议题、拉取请求或提交的评论](/articles/reporting-abuse-or-spam)时触发。{% endif %}
+| `show_private_contributions_count` | Triggered when you [publicize private contributions on your profile](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile).{% if currentVersion != "github-ae@latest" %}
+| `two_factor_requested` | 当 {% data variables.product.product_name %} 要求您提供[双重身份验证代码](/articles/accessing-github-using-two-factor-authentication)时触发。{% endif %}
-### 导出安全日志
+#### `user_status` category actions
+
+| 操作 | 描述 |
+| --------- | -------------------------------------------------------------------------------------------- |
+| `update` | 当您在个人资料中设置或更改状态时触发。 更多信息请参阅“[设置状态](/articles/personalizing-your-profile/#setting-a-status)”。 |
+| `destroy` | 当您在个人资料中清除状态时触发。 |
-{% data reusables.audit_log.export-log %}
-{% data reusables.audit_log.exported-log-keys-and-values %}
-{% endif %}
diff --git a/translations/zh-CN/content/github/building-a-strong-community/about-issue-and-pull-request-templates.md b/translations/zh-CN/content/github/building-a-strong-community/about-issue-and-pull-request-templates.md
index 296183e382b2..c3e3530ccd23 100644
--- a/translations/zh-CN/content/github/building-a-strong-community/about-issue-and-pull-request-templates.md
+++ b/translations/zh-CN/content/github/building-a-strong-community/about-issue-and-pull-request-templates.md
@@ -11,7 +11,11 @@ versions:
在仓库中创建议题和拉取请求后,贡献者可以根据仓库的参与指南使用模板打开议题或描述其拉取请求中提议的更改。 有关向仓库添加参与指南的更多信息,请参阅“[设置仓库贡献者指南](/articles/setting-guidelines-for-repository-contributors)”。
-您可以为组织{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %} 或用户帐户{% endif %} 创建默认的议题和拉取请求模板。 更多信息请参阅“[创建默认社区健康文件](/github/building-a-strong-community/creating-a-default-community-health-file)”。
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+You can create default issue and pull request templates for your organization or user account. 更多信息请参阅“[创建默认社区健康文件](/github/building-a-strong-community/creating-a-default-community-health-file)”。
+
+{% endif %}
### 议题模板
diff --git a/translations/zh-CN/content/github/building-a-strong-community/about-wikis.md b/translations/zh-CN/content/github/building-a-strong-community/about-wikis.md
index 74974878d3bc..ee109b9c7e59 100644
--- a/translations/zh-CN/content/github/building-a-strong-community/about-wikis.md
+++ b/translations/zh-CN/content/github/building-a-strong-community/about-wikis.md
@@ -15,9 +15,9 @@ versions:
使用 wiki,可以像在 {% data variables.product.product_name %} 的任何其他位置一样编写内容。 更多信息请参阅“[在 {% data variables.product.prodname_dotcom %} 上编写和设置格式](/articles/getting-started-with-writing-and-formatting-on-github)”。 我们使用[开源标记库](https://github.com/github/markup)将不同的格式转换为 HTML,以便选择使用 Markdown 或任何其他支持的格式编写。
-Wikis 可在公共仓库中公开,也可在私有仓库中限于对仓库具有访问权限的人访问。 更多信息请参阅“[设置仓库可见性](/articles/setting-repository-visibility)”。
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}If you create a wiki in a public repository, the wiki is available to {% if enterpriseServerVersions contains currentVersion %}anyone with access to {% data variables.product.product_location %}{% else %}the public{% endif %}. {% endif %}If you create a wiki in an internal or private repository, {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}people{% elsif currentVersion == "github-ae@latest" %}enterprise members{% endif %} with access to the repository can also access the wiki. 更多信息请参阅“[设置仓库可见性](/articles/setting-repository-visibility)”。
-您可以直接在 {% data variables.product.product_name %} 上编辑 wikis,也可在本地编辑 wiki 文件。 默认情况下,只有能够写入仓库的人才可更改 wikis,但您可以允许 {% data variables.product.product_name %} 上的每个人参与公共仓库中的 wiki。 更多信息请参阅“[更改 wikis 的访问权限](/articles/changing-access-permissions-for-wikis)”。
+您可以直接在 {% data variables.product.product_name %} 上编辑 wikis,也可在本地编辑 wiki 文件。 By default, only people with write access to your repository can make changes to wikis, although you can allow everyone on {% data variables.product.product_location %} to contribute to a wiki in {% if currentVersion == "github-ae@latest" %}an internal{% else %}a public{% endif %} repository. 更多信息请参阅“[更改 wikis 的访问权限](/articles/changing-access-permissions-for-wikis)”。
### 延伸阅读
diff --git a/translations/zh-CN/content/github/building-a-strong-community/adding-a-license-to-a-repository.md b/translations/zh-CN/content/github/building-a-strong-community/adding-a-license-to-a-repository.md
index cc8e3d6deb29..b0a78164b246 100644
--- a/translations/zh-CN/content/github/building-a-strong-community/adding-a-license-to-a-repository.md
+++ b/translations/zh-CN/content/github/building-a-strong-community/adding-a-license-to-a-repository.md
@@ -6,7 +6,6 @@ redirect_from:
versions:
free-pro-team: '*'
enterprise-server: '*'
- github-ae: '*'
---
如果在仓库中包含可检测的许可,仓库的访问者将会在仓库页面顶部看到它。 要阅读整个许可文件,请单击许可名称。
diff --git a/translations/zh-CN/content/github/building-a-strong-community/adding-support-resources-to-your-project.md b/translations/zh-CN/content/github/building-a-strong-community/adding-support-resources-to-your-project.md
index 5aff7dc3d223..5532cee13dac 100644
--- a/translations/zh-CN/content/github/building-a-strong-community/adding-support-resources-to-your-project.md
+++ b/translations/zh-CN/content/github/building-a-strong-community/adding-support-resources-to-your-project.md
@@ -13,7 +13,11 @@ versions:

-您可以为组织{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %} 或用户帐户{% endif %} 创建默认的支持资源。 更多信息请参阅“[创建默认社区健康文件](/github/building-a-strong-community/creating-a-default-community-health-file)”。
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+You can create default support resources for your organization or user account. 更多信息请参阅“[创建默认社区健康文件](/github/building-a-strong-community/creating-a-default-community-health-file)”。
+
+{% endif %}
{% tip %}
diff --git a/translations/zh-CN/content/github/building-a-strong-community/configuring-issue-templates-for-your-repository.md b/translations/zh-CN/content/github/building-a-strong-community/configuring-issue-templates-for-your-repository.md
index 676eb16f608c..77af71f137e7 100644
--- a/translations/zh-CN/content/github/building-a-strong-community/configuring-issue-templates-for-your-repository.md
+++ b/translations/zh-CN/content/github/building-a-strong-community/configuring-issue-templates-for-your-repository.md
@@ -10,10 +10,16 @@ versions:
github-ae: '*'
---
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
{% data reusables.repositories.default-issue-templates %}
+{% endif %}
+
{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %}
+
### 创建议题模板
+
{% endif %}
{% data reusables.repositories.navigate-to-repo %}
diff --git a/translations/zh-CN/content/github/building-a-strong-community/creating-a-default-community-health-file.md b/translations/zh-CN/content/github/building-a-strong-community/creating-a-default-community-health-file.md
index ffee368355c9..f309686e706b 100644
--- a/translations/zh-CN/content/github/building-a-strong-community/creating-a-default-community-health-file.md
+++ b/translations/zh-CN/content/github/building-a-strong-community/creating-a-default-community-health-file.md
@@ -7,7 +7,6 @@ redirect_from:
versions:
free-pro-team: '*'
enterprise-server: '*'
- github-ae: '*'
---
### 关于默认社区健康文件
diff --git a/translations/zh-CN/content/github/building-a-strong-community/creating-a-pull-request-template-for-your-repository.md b/translations/zh-CN/content/github/building-a-strong-community/creating-a-pull-request-template-for-your-repository.md
index a02aace2b136..2d7b6d821253 100644
--- a/translations/zh-CN/content/github/building-a-strong-community/creating-a-pull-request-template-for-your-repository.md
+++ b/translations/zh-CN/content/github/building-a-strong-community/creating-a-pull-request-template-for-your-repository.md
@@ -13,7 +13,11 @@ versions:
您可以在任何支持的文件夹中创建 *PULL_REQUEST_TEMPLATE/* 子目录,以包含多个拉取请求模板,并使用 `template` 查询参数指定填充拉取请求正文的模板。 更多信息请参阅“[关于使用查询参数自动化议题和拉取请求](/articles/about-automation-for-issues-and-pull-requests-with-query-parameters)”。
-您可以为组织{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %} 或用户帐户{% endif %} 创建默认的拉取请求模板。 更多信息请参阅“[创建默认社区健康文件](/github/building-a-strong-community/creating-a-default-community-health-file)”。
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+您可以为组织{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" %} 或用户帐户{% endif %} 创建默认的拉取请求模板。 更多信息请参阅“[创建默认社区健康文件](/github/building-a-strong-community/creating-a-default-community-health-file)”。
+
+{% endif %}
### 添加拉取请求模板
diff --git a/translations/zh-CN/content/github/building-a-strong-community/locking-conversations.md b/translations/zh-CN/content/github/building-a-strong-community/locking-conversations.md
index 215ea8a284ef..ac124a998380 100644
--- a/translations/zh-CN/content/github/building-a-strong-community/locking-conversations.md
+++ b/translations/zh-CN/content/github/building-a-strong-community/locking-conversations.md
@@ -15,7 +15,7 @@ versions:

-当对话锁定时,仅[具有写入权限的人员](/articles/repository-permission-levels-for-an-organization/)以及[仓库所有者和协作者](/articles/permission-levels-for-a-user-account-repository/#collaborator-access-on-a-repository-owned-by-a-user-account)才可添加、隐藏和删除评论。
+当对话锁定时,仅[具有写入权限的人员](/articles/repository-permission-levels-for-an-organization/)以及[仓库所有者和协作者](/articles/permission-levels-for-a-user-account-repository/#collaborator-access-for-a-repository-owned-by-a-user-account)才可添加、隐藏和删除评论。
要搜索仓库中未存档的已锁定对话,可以使用搜索限定符 `is:locked` 和 `archived:false`。 对话在存档的仓库中会自动锁定。 更多信息请参阅“[搜索议题和拉取请求](/articles/searching-issues-and-pull-requests#search-based-on-whether-a-conversation-is-locked)”。
diff --git a/translations/zh-CN/content/github/building-a-strong-community/manually-creating-a-single-issue-template-for-your-repository.md b/translations/zh-CN/content/github/building-a-strong-community/manually-creating-a-single-issue-template-for-your-repository.md
index 6b3e04d02faf..9d59afea69f9 100644
--- a/translations/zh-CN/content/github/building-a-strong-community/manually-creating-a-single-issue-template-for-your-repository.md
+++ b/translations/zh-CN/content/github/building-a-strong-community/manually-creating-a-single-issue-template-for-your-repository.md
@@ -39,8 +39,12 @@ assignees: octocat
{% endif %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
{% data reusables.repositories.default-issue-templates %}
+{% endif %}
+
### 添加议题模板
{% data reusables.repositories.navigate-to-repo %}
diff --git a/translations/zh-CN/content/github/building-a-strong-community/setting-guidelines-for-repository-contributors.md b/translations/zh-CN/content/github/building-a-strong-community/setting-guidelines-for-repository-contributors.md
index 8d9264108f1d..954ca4085ee5 100644
--- a/translations/zh-CN/content/github/building-a-strong-community/setting-guidelines-for-repository-contributors.md
+++ b/translations/zh-CN/content/github/building-a-strong-community/setting-guidelines-for-repository-contributors.md
@@ -20,7 +20,11 @@ versions:
对于所有者和参与者来说,参与指南节省了由于不正确创建必须拒绝和重新提交的拉取请求或议题而导致的时间和麻烦。
-您可以为组织{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %} 或用户帐户{% endif %} 创建默认的参与指南。 更多信息请参阅“[创建默认社区健康文件](/github/building-a-strong-community/creating-a-default-community-health-file)”。
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+您可以为组织{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" %} 或用户帐户{% endif %} 创建默认的参与指南。 更多信息请参阅“[创建默认社区健康文件](/github/building-a-strong-community/creating-a-default-community-health-file)”。
+
+{% endif %}
{% tip %}
@@ -53,5 +57,5 @@ versions:
### 延伸阅读
- 开源指南的“[启动开源项目](https://opensource.guide/starting-a-project/)”部分{% if currentVersion == "free-pro-team@latest" %}
-- [{% data variables.product.prodname_learning %}]({% data variables.product.prodname_learning_link %}){% endif %}
-- "[添加许可到仓库](/articles/adding-a-license-to-a-repository)"
+- [{% data variables.product.prodname_learning %}]({% data variables.product.prodname_learning_link %}){% endif %}{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- "[Adding a license to a repository](/articles/adding-a-license-to-a-repository)"{% endif %}
diff --git a/translations/zh-CN/content/github/collaborating-with-issues-and-pull-requests/about-branches.md b/translations/zh-CN/content/github/collaborating-with-issues-and-pull-requests/about-branches.md
index 92a6dba43e77..5d9001a375ef 100644
--- a/translations/zh-CN/content/github/collaborating-with-issues-and-pull-requests/about-branches.md
+++ b/translations/zh-CN/content/github/collaborating-with-issues-and-pull-requests/about-branches.md
@@ -23,7 +23,7 @@ versions:
### 关于默认分支
-{% data reusables.branches.new-repo-default-branch %} 默认分支是任何人访问您的仓库时 {% data variables.product.prodname_dotcom %} 显示的分支。 默认分支也是初始分支,当有人克隆存储库时,Git 会在本地检出该分支。 {% data reusables.branches.default-branch-automatically-base-branch %}
+{% data reusables.branches.new-repo-default-branch %} 默认分支是任何人访问您的仓库时 {% data variables.product.prodname_dotcom %} 显示的分支。 The default branch is also the initial branch that Git checks out locally when someone clones the repository. {% data reusables.branches.default-branch-automatically-base-branch %}
默认情况下,{% data variables.product.product_name %} 将任何新仓库中的默认分支命名为{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}`main`{% else %}`master`{% endif %}。
@@ -75,7 +75,7 @@ versions:
- 如果对分支启用了必需拉取请求审查,则在满足拉取请求审查策略中的所有要求之前,无法将更改合并到分支。 更多信息请参阅“[合并拉取请求](/articles/merging-a-pull-request)”。
- 如果对分支启用了代码所有者的必需审查,并且拉取请求修改具有所有者的代码,则代码所有者必须批准拉取请求后才可合并。 更多信息请参阅“[关于代码所有者](/articles/about-code-owners)”。
- 如果对分支启用了必需提交签名,则无法将任何提交推送到未签名和验证的分支。 更多信息请参阅“[关于提交签名验证](/articles/about-commit-signature-verification)”和“[关于必需提交签名](/articles/about-required-commit-signing)”。{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
-- 如果您使用 {% data variables.product.prodname_dotcom %} 的冲突编辑器来解决从受保护分支创建拉取请求的冲突,{% data variables.product.prodname_dotcom %} 可帮助您为拉取请求创建一个备用分支,以解决合并冲突。 更多信息请参阅“[解决 {% data variables.product.prodname_dotcom %} 上的合并冲突](/github/collaborating-with-issues-and-pull-requests/resolving-a-merge-conflict-on-github)”。{% endif %}
+- If you use {% data variables.product.prodname_dotcom %}'s conflict editor to fix conflicts for a pull request that you created from a protected branch, {% data variables.product.prodname_dotcom %} helps you to create an alternative branch for the pull request, so that your resolution of the conflicts can be merged. 更多信息请参阅“[解决 {% data variables.product.prodname_dotcom %} 上的合并冲突](/github/collaborating-with-issues-and-pull-requests/resolving-a-merge-conflict-on-github)”。{% endif %}
### 延伸阅读
diff --git a/translations/zh-CN/content/github/collaborating-with-issues-and-pull-requests/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility.md b/translations/zh-CN/content/github/collaborating-with-issues-and-pull-requests/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility.md
index 8b8de1a8c056..8d1d9c21df1c 100644
--- a/translations/zh-CN/content/github/collaborating-with-issues-and-pull-requests/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility.md
+++ b/translations/zh-CN/content/github/collaborating-with-issues-and-pull-requests/what-happens-to-forks-when-a-repository-is-deleted-or-changes-visibility.md
@@ -16,14 +16,20 @@ versions:
当您删除私有仓库时,其所有私有复刻也将被删除。
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
#### 删除公共仓库
当您删除公共仓库时,将选择现有的公共复刻之一作为新的父仓库。 所有其他仓库均从这一新的父仓库复刻,并且后续的拉取请求都转到这一新的父仓库。
+{% endif %}
+
#### 私有复刻和权限
{% data reusables.repositories.private_forks_inherit_permissions %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
#### 将公共仓库更改为私有仓库
如果将公共仓库设为私有,其公共复刻将拆分到新网络中。 与删除公共仓库一样,选择现有的公共分支之一作为新的父仓库,并且所有其他仓库都从这个新的父仓库中复刻。 后续的拉取请求都转到这一新的父仓库。
@@ -46,9 +52,30 @@ versions:
如果将私有仓库设为公共然后删除,其私有复刻将作为单独网络中的独立私有仓库继续存在。
+{% endif %}
+
+{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.19" %}
+
+#### Changing the visibility of an internal repository
+
+{% note %}
+
+**注:**{% data reusables.gated-features.internal-repos %}
+
+{% endnote %}
+
+If the policy for your enterprise permits forking, any fork of an internal repository will be private. If you change the visibility of an internal repository, any fork owned by an organization or user account will remain private.
+
+##### Deleting the internal repository
+
+If you change the visibility of an internal repository and then delete the repository, the forks will continue to exist in a separate network.
+
+{% endif %}
+
### 延伸阅读
- “[设置仓库可见性](/articles/setting-repository-visibility)”
- "[关于复刻](/articles/about-forks)"
- "[管理仓库的复刻策略](/github/administering-a-repository/managing-the-forking-policy-for-your-repository)"
- "[管理组织的复刻策略](/github/setting-up-and-managing-organizations-and-teams/managing-the-forking-policy-for-your-organization)"
+- "{% if currentVersion == "free-pro-team@latest" %}[Enforcing repository management policies in your enterprise account](/github/setting-up-and-managing-your-enterprise/enforcing-repository-management-policies-in-your-enterprise-account#enforcing-a-policy-on-forking-private-or-internal-repositories){% else %}[Enforcing repository management policies in your enterprise](/admin/policies/enforcing-repository-management-policies-in-your-enterprise#enforcing-a-policy-on-forking-private-or-internal-repositories){% endif %}"
diff --git a/translations/zh-CN/content/github/creating-cloning-and-archiving-repositories/about-readmes.md b/translations/zh-CN/content/github/creating-cloning-and-archiving-repositories/about-readmes.md
index 844698dd60bb..90a03a1166a4 100644
--- a/translations/zh-CN/content/github/creating-cloning-and-archiving-repositories/about-readmes.md
+++ b/translations/zh-CN/content/github/creating-cloning-and-archiving-repositories/about-readmes.md
@@ -11,7 +11,11 @@ versions:
github-ae: '*'
---
-自述文件连同 {% if currentVersion == "free-pro-team@latest" %}[仓库许可证](/articles/licensing-a-repository)、[参与指南](/articles/setting-guidelines-for-repository-contributors)和[行为准则](/articles/adding-a-code-of-conduct-to-your-project){% else %}、[仓库许可证](/articles/licensing-a-repository)和[参与指南](/articles/setting-guidelines-for-repository-contributors){% endif %}一起,帮助您,沟通项目要求以及管理对项目的参与。
+### 关于自述文件
+
+You can add a README file to a repository to communicate important information about your project. A README, along with a repository license{% if currentVersion == "free-pro-team@latest" %}, contribution guidelines, and a code of conduct{% elsif enterpriseServerVersions contains currentVersion %} and contribution guidelines{% endif %}, communicates expectations for your project and helps you manage contributions.
+
+For more information about providing guidelines for your project, see {% if currentVersion == "free-pro-team@latest" %}"[Adding a code of conduct to your project](/github/building-a-strong-community/adding-a-code-of-conduct-to-your-project)" and {% endif %}"[Setting up your project for healthy contributions](/github/building-a-strong-community/setting-up-your-project-for-healthy-contributions)."
自述文件通常是访问者在访问仓库时看到的第一个项目。 自述文件通常包含以下信息:
- 项目做什么
@@ -26,8 +30,12 @@ versions:
{% if currentVersion == "free-pro-team@latest" or currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21"%}
+
{% data reusables.profile.profile-readme %}
+{% endif %}
+

{% endif %}
diff --git a/translations/zh-CN/content/github/creating-cloning-and-archiving-repositories/licensing-a-repository.md b/translations/zh-CN/content/github/creating-cloning-and-archiving-repositories/licensing-a-repository.md
index f75d642ac574..9274ba154fc1 100644
--- a/translations/zh-CN/content/github/creating-cloning-and-archiving-repositories/licensing-a-repository.md
+++ b/translations/zh-CN/content/github/creating-cloning-and-archiving-repositories/licensing-a-repository.md
@@ -7,7 +7,6 @@ redirect_from:
versions:
free-pro-team: '*'
enterprise-server: '*'
- github-ae: '*'
---
### 选择合适的许可
@@ -18,7 +17,7 @@ versions:
{% note %}
-**注:**如果您在 GitHub 的公共仓库中发布源代码,{% if currentVersion == "free-pro-team@latest" %}根据[服务条款](/articles/github-terms-of-service),{% endif %}其他 GitHub 用户有权利在 GitHub 站点中查看您的仓库并对其复刻。 如果您已创建公共仓库,并且不再希望用户访问它,便可将仓库设为私有。 在将公共仓库转换为私有仓库时,其他用户创建的现有复刻或本地副本仍将存在。 更多信息请参阅“[将公共仓库设为私有](/articles/making-a-public-repository-private)”。
+**Note:** If you publish your source code in a public repository on {% data variables.product.product_name %}, {% if currentVersion == "free-pro-team@latest" %}according to the [Terms of Service](/articles/github-terms-of-service), {% endif %}other users of {% data variables.product.product_location %} have the right to view and fork your repository. If you have already created a repository and no longer want users to have access to the repository, you can make the repository private. When you change the visibility of a repository to private, existing forks or local copies created by other users will still exist. 更多信息请参阅“[设置仓库可见性](/github/administering-a-repository/setting-repository-visibility)”。
{% endnote %}
diff --git a/translations/zh-CN/content/github/developing-online-with-codespaces/using-codespaces-in-visual-studio.md b/translations/zh-CN/content/github/developing-online-with-codespaces/using-codespaces-in-visual-studio.md
index 09bb634fa2a7..27efd7e74b58 100644
--- a/translations/zh-CN/content/github/developing-online-with-codespaces/using-codespaces-in-visual-studio.md
+++ b/translations/zh-CN/content/github/developing-online-with-codespaces/using-codespaces-in-visual-studio.md
@@ -46,8 +46,12 @@ versions:
### 配置 {% data variables.product.prodname_vs %} 的代码空间
-通过 {% data variables.product.prodname_vs %} 创建的默认代码空间环境包括流行的框架和工具,例如 .NET Core、Microsoft SQL Server、Python 和 Windows SDK。 使用 {% data variables.product.prodname_vs %} 创建的{% data variables.product.prodname_github_codespaces %} 可通过一组 `devcontainers.json` 属性和 {% data variables.product.prodname_vs %} 随附的新工具 devinit 进行自定义。
+A codespace, created with {% data variables.product.prodname_vs %}, can be customized through a new tool called devinit, a command line tool included with {% data variables.product.prodname_vs %}.
#### devinit
-[devinit](https://docs.microsoft.com/visualstudio/devinit/getting-started-with-devinit) 命令行工具允许您将额外的框架和工具安装到 Windows 开发代码空间中,以及运行 PowerShell 脚本或修改环境变量。 devinit 支持名为 [devinit.json](https://docs.microsoft.com/visualstudio/devinit/devinit-json) 的配置文件,该文件可添加到您的项目中用于创建自定义和可重复的开发环境。 有关 Windows 代码空间配置和 devinit 的更多信息,请参阅 {% data variables.product.prodname_vs %} 文档中的[自定义代码空间](https://docs.microsoft.com/visualstudio/ide/codespaces/customize-codespaces)。
+[devinit](https://docs.microsoft.com/visualstudio/devinit/getting-started-with-devinit) lets you install additional frameworks and tools into your Windows development codespaces, modify environment variables, and more.
+
+devinit supports a configuration file called [devinit.json](https://docs.microsoft.com/visualstudio/devinit/devinit-json). You can add this file to your project if you want to create a customized and repeatable development environment. When you use devinit with a [devcontainer.json](https://docs.microsoft.com/visualstudio/ide/codespaces/customize-codespaces#running-devinit-when-creating-a-codespace) file, your codespaces will be automatically configured on creation.
+
+有关 Windows 代码空间配置和 devinit 的更多信息,请参阅 {% data variables.product.prodname_vs %} 文档中的[自定义代码空间](https://docs.microsoft.com/visualstudio/ide/codespaces/customize-codespaces)。 For more information about devinit, see [Getting started with devinit](https://docs.microsoft.com/visualstudio/devinit/getting-started-with-devinit).
diff --git a/translations/zh-CN/content/github/getting-started-with-github/create-a-repo.md b/translations/zh-CN/content/github/getting-started-with-github/create-a-repo.md
index f6af5668ec8f..e3e39f8746b8 100644
--- a/translations/zh-CN/content/github/getting-started-with-github/create-a-repo.md
+++ b/translations/zh-CN/content/github/getting-started-with-github/create-a-repo.md
@@ -10,14 +10,26 @@ versions:
github-ae: '*'
---
+{% if currentVersion == "free-pro-team@latest" %}
+
您可以在 {% data variables.product.product_name %} 仓库中存储各种项目,包括开源项目。 通过[开源项目](http://opensource.org/about),您可以共享代码以开发更好、更可靠的软件。
+{% elsif enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
+
+You can store a variety of projects in {% data variables.product.product_name %} repositories, including innersource projects. With innersource, you can share code to make better, more reliable software. For more information on innersource, see {% data variables.product.company_short %}'s whitepaper "[An introduction to innersource](https://resources.github.com/whitepapers/introduction-to-innersource/)."
+
+{% endif %}
+
+{% if currentVersion == "free-pro-team@latest" %}
+
{% note %}
**注:**您可以为开源项目创建公共仓库。 创建公共仓库时,请确保包含[许可文件](http://choosealicense.com/)以确定您希望与其他人共享项目。 {% data reusables.open-source.open-source-guide-repositories %} {% data reusables.open-source.open-source-learning-lab %}
{% endnote %}
+{% endif %}
+
{% data reusables.repositories.create_new %}
2. 为仓库键入简短、令人难忘的名称。 例如 "hello-world"。 
3. (可选)添加仓库的说明。 For example, "My first repository on
diff --git a/translations/zh-CN/content/github/getting-started-with-github/fork-a-repo.md b/translations/zh-CN/content/github/getting-started-with-github/fork-a-repo.md
index c511f7524b12..9f0346537a92 100644
--- a/translations/zh-CN/content/github/getting-started-with-github/fork-a-repo.md
+++ b/translations/zh-CN/content/github/getting-started-with-github/fork-a-repo.md
@@ -25,10 +25,16 @@ versions:
开源软件的理念是通过共享代码,可以开发出更好、更可靠的软件。 更多信息请参阅 Open Source Initiative(开源倡议)上的“[关于开源倡议](http://opensource.org/about)”。
+For more information about applying open source principles to your organization's development work on {% data variables.product.product_location %}, see {% data variables.product.prodname_dotcom %}'s whitepaper "[An introduction to innersource](https://resources.github.com/whitepapers/introduction-to-innersource/)."
+
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
从其他人的项目复刻创建公共仓库时,请确保包含许可文件以确定您希望与其他人共享项目。 更多信息请参阅 choosealicense 上的“[选择开源许可](http://choosealicense.com/)”。
{% data reusables.open-source.open-source-guide-repositories %} {% data reusables.open-source.open-source-learning-lab %}
+{% endif %}
+
{% note %}
**注**:{% data reusables.repositories.desktop-fork %}
diff --git a/translations/zh-CN/content/github/getting-started-with-github/setting-up-a-trial-of-github-enterprise-server.md b/translations/zh-CN/content/github/getting-started-with-github/setting-up-a-trial-of-github-enterprise-server.md
index add90be06adb..7dd422d93ede 100644
--- a/translations/zh-CN/content/github/getting-started-with-github/setting-up-a-trial-of-github-enterprise-server.md
+++ b/translations/zh-CN/content/github/getting-started-with-github/setting-up-a-trial-of-github-enterprise-server.md
@@ -36,7 +36,7 @@ versions:
- [{% data variables.product.prodname_dotcom %} 快速入门指南](https://resources.github.com/webcasts/Quick-start-guide-to-GitHub/)网络直播
- {% data variables.product.prodname_dotcom %} 指南中的[了解 {% data variables.product.prodname_dotcom %} 流程](https://guides.github.com/introduction/flow/)
- {% data variables.product.prodname_dotcom %} 指南中的 [Hello World](https://guides.github.com/activities/hello-world/)
-3. To configure your instance to meet your organization's needs, see "[Configuring your enterprise](/admin/configuration/configuring-your-enterprise)."
+3. To configure your instance to meet your organization's needs, see "[Configuring your enterprise](/enterprise/admin/configuration/configuring-your-enterprise)."
4. 要将 {% data variables.product.prodname_ghe_server %} 与您的身份提供程序集成,请参阅“[使用 SAML](/enterprise/admin/user-management/using-saml)”和“[使用 LDAP](/enterprise/admin/authentication/using-ldap)”。
5. 邀请不限数量的人员加入您的试用版。
- 使用内置身份验证或配置的身份提供程序将用户添加到 {% data variables.product.prodname_ghe_server %} 实例。 更多信息请参阅“[使用内置身份验证](/enterprise/admin/user-management/using-built-in-authentication)”。
diff --git a/translations/zh-CN/content/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies.md b/translations/zh-CN/content/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies.md
index c0f46c36576b..a628b146fd7f 100644
--- a/translations/zh-CN/content/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies.md
+++ b/translations/zh-CN/content/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies.md
@@ -71,7 +71,7 @@ When {% data variables.product.product_name %} identifies a vulnerable dependenc
You can see all of the alerts that affect a particular project{% if currentVersion == "free-pro-team@latest" %} on the repository's Security tab or{% endif %} in the repository's dependency graph.{% if currentVersion == "free-pro-team@latest" %} For more information, see "[Viewing and updating vulnerable dependencies in your repository](/articles/viewing-and-updating-vulnerable-dependencies-in-your-repository)."{% endif %}
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
-By default, we notify people with admin permissions in the affected repositories about new {% data variables.product.prodname_dependabot_alerts %}.{% endif %} {% if currentVersion == "free-pro-team@latest" %}{% data variables.product.product_name %} never publicly discloses identified vulnerabilities for any repository. You can also make {% data variables.product.prodname_dependabot_alerts %} visible to additional people or teams working repositories that you own or have admin permissions for. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-dependabot-alerts)."
+By default, we notify people with admin permissions in the affected repositories about new {% data variables.product.prodname_dependabot_alerts %}.{% endif %} {% if currentVersion == "free-pro-team@latest" %}{% data variables.product.product_name %} never publicly discloses identified vulnerabilities for any repository. You can also make {% data variables.product.prodname_dependabot_alerts %} visible to additional people or teams working repositories that you own or have admin permissions for. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-security-alerts)."
{% endif %}
{% if enterpriseServerVersions contains currentVersion and currentVersion ver_lt "enterprise-server@2.22" %}
diff --git a/translations/zh-CN/content/github/managing-subscriptions-and-notifications-on-github/about-notifications.md b/translations/zh-CN/content/github/managing-subscriptions-and-notifications-on-github/about-notifications.md
index 1e143ee7e80b..3a76cf39214e 100644
--- a/translations/zh-CN/content/github/managing-subscriptions-and-notifications-on-github/about-notifications.md
+++ b/translations/zh-CN/content/github/managing-subscriptions-and-notifications-on-github/about-notifications.md
@@ -21,7 +21,12 @@ versions:
- 关于特定议题、拉取请求或 Gist 的对话。
- 仓库或团队讨论中的所有活动。
- CI 活动,例如仓库中使用 {% data variables.product.prodname_actions %} 设置的工作流程的状态。
+{% if currentVersion == "free-pro-team@latest" %}
+- Issues, pulls requests, releases and discussions (if enabled) in a repository.
+{% endif %}
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}
- 仓库中的发行版。
+{% endif %}
您也可以选择自动关注所有您有推送访问权限的仓库,但复刻除外。 您可以通过单击 **Watch(关注)**来手动关注您有权访问的任何其他仓库。
diff --git a/translations/zh-CN/content/github/managing-subscriptions-and-notifications-on-github/configuring-notifications.md b/translations/zh-CN/content/github/managing-subscriptions-and-notifications-on-github/configuring-notifications.md
index a7a69ebaafc9..0898413f48ff 100644
--- a/translations/zh-CN/content/github/managing-subscriptions-and-notifications-on-github/configuring-notifications.md
+++ b/translations/zh-CN/content/github/managing-subscriptions-and-notifications-on-github/configuring-notifications.md
@@ -21,16 +21,17 @@ versions:
### 通知递送选项
-您有三个基本的通知递送选项:
- - the notifications inbox on {% data variables.product.product_name %}{% if currentVersion == "free-pro-team@latest" %}
- - {% data variables.product.prodname_mobile %} 上的通知收件箱,它与 {% data variables.product.product_name %} 上的收件箱同步{% endif %}
- - an email client that uses a verified email address, which can also sync with the notifications inbox on {% data variables.product.product_name %}{% if currentVersion == "free-pro-team@latest" %} and {% data variables.product.prodname_mobile %}{% endif %}
+You can receive notifications for activity on {% data variables.product.product_name %} in the following locations.
+
+ - The notifications inbox in the {% data variables.product.product_name %} web interface{% if currentVersion == "free-pro-team@latest" %}
+ - The notifications inbox on {% data variables.product.prodname_mobile %}, which syncs with the inbox on {% data variables.product.product_name %}{% endif %}
+ - An email client that uses a verified email address, which can also sync with the notifications inbox on {% data variables.product.product_name %}{% if currentVersion == "free-pro-team@latest" %} and {% data variables.product.prodname_mobile %}{% endif %}
{% if currentVersion == "free-pro-team@latest" %}
{% data reusables.notifications-v2.notifications-inbox-required-setting %} 更多信息请参阅“[选择通知设置](#choosing-your-notification-settings)”。
{% endif %}
-{% data reusables.notifications-v2.tip-for-syncing-email-and-your-inbox-on-github %}
+{% data reusables.notifications.shared_state %}
#### 通知收件箱的优点
@@ -59,8 +60,21 @@ In addition, the notifications inbox on
### 关于参与和查看通知
-关注仓库,意味着订阅该仓库中的活动更新。 同样,关注特定团队的讨论,意味着订阅该团队页面上的所有对话更新。 要查看您关注的仓库,请参阅 [https://github.com/watching](https://github.com/watching)。 更多信息请参阅“[在 GitHub 上管理订阅和通知](/github/managing-subscriptions-and-notifications-on-github/managing-subscriptions-for-activity-on-github)”。
+关注仓库,意味着订阅该仓库中的活动更新。 同样,关注特定团队的讨论,意味着订阅该团队页面上的所有对话更新。 更多信息请参阅“[关于团队讨论](/github/building-a-strong-community/about-team-discussions)”。
+
+To see repositories that you're watching, go to your [watching page](https://github.com/watching). 更多信息请参阅“[在 GitHub 上管理订阅和通知](/github/managing-subscriptions-and-notifications-on-github/managing-subscriptions-for-activity-on-github)”。
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}
+#### 配置通知
+{% endif %}
+You can configure notifications for a repository on the repository page, or on your watching page.
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %} You can choose to only receive notifications for releases in a repository, or ignore all notifications for a repository.{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+
+#### About custom notifications
+{% data reusables.notifications-v2.custom-notifications-beta %}
+You can customize notifications for a repository, for example, you can choose to only be notified when updates to one or more types of events (issues, pull request, releases, discussions) happen within a repository, or ignore all notifications for a repository.
+{% endif %} For more information, see "[Viewing your subscriptions](/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions#configuring-your-watch-settings-for-an-individual-repository)."
+#### Participating in conversations
每当您在对话中发表评论或有人 @提及您的用户名时,您都在_参与_对话。 默认情况下,当您参与对话时,会自动订阅该对话。 您可以通过单击议题或拉取请求上的 **Unsubscribe(取消订阅)**或通过通知收件箱中的 **Unsubscribe(取消订阅)**选项,手动取消订阅已参与的对话。
For conversations you're watching or participating in, you can choose whether you want to receive notifications by email or through the notifications inbox on {% data variables.product.product_name %}{% if currentVersion == "free-pro-team@latest" %} and {% data variables.product.prodname_mobile %}{% endif %}.
@@ -95,7 +109,9 @@ If you do not enable watching or participating notifications for web{% if curren
- 拉取请求推送。
- 您自己的更新,例如当您打开、评论或关闭议题或拉取请求时。
-您还可以向特定仓库的不同电子邮件地址发送通知,具体取决于拥有仓库的组织。 例如,您可以向经验证的个人电子邮件地址发送特定公共仓库的通知。 您的组织可能要求验证特定域的电子邮件地址。 更多信息请参阅“[选择接收组织的电子邮件通知的位置](/github/managing-subscriptions-and-notifications-on-github/configuring-notifications#choosing-where-your-organizations-email-notifications-are-sent)”。
+Depending on the organization that owns the repository, you can also send notifications to different email addresses. 您的组织可能要求验证特定域的电子邮件地址。 For more information, see "[Choosing where your organization’s email notifications are sent](/github/managing-subscriptions-and-notifications-on-github/configuring-notifications#choosing-where-your-organizations-email-notifications-are-sent)."
+
+You can also send notifications for a specific repository to an email address. 更多信息请参阅“[关于推送到仓库的电子邮件通知](/github/administering-a-repository/about-email-notifications-for-pushes-to-your-repository)。”
{% data reusables.notifications-v2.email-notification-caveats %}
diff --git a/translations/zh-CN/content/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions.md b/translations/zh-CN/content/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions.md
index 1f9b6e653ae1..bc261baf49a5 100644
--- a/translations/zh-CN/content/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions.md
+++ b/translations/zh-CN/content/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions.md
@@ -55,6 +55,13 @@ versions:
{% data reusables.notifications.access_notifications %}
1. 在左侧边栏中的仓库列表下,使用“Manage notifications(管理通知)”下拉按钮单击 **Watched repositories(已关注的仓库)**。 
2. 在关注的仓库页面上,评估您关注的仓库后,选择是否:
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}
- 取消关注仓库
- 只关注某仓库的发行版
- 忽略某仓库的所有通知
+{% endif %}
+{% if currentVersion == "free-pro-team@latest" %}
+ - 取消关注仓库
+ - 忽略某仓库的所有通知
+ - customize the types of event you receive notifications for (issues, pull requests, releases or discussions, if enabled)
+{% endif %}
\ No newline at end of file
diff --git a/translations/zh-CN/content/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions.md b/translations/zh-CN/content/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions.md
index ccfd2295f580..0372f1e18450 100644
--- a/translations/zh-CN/content/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions.md
+++ b/translations/zh-CN/content/github/managing-subscriptions-and-notifications-on-github/viewing-your-subscriptions.md
@@ -32,7 +32,16 @@ versions:
更多信息请参阅“[配置通知](/github/managing-subscriptions-and-notifications-on-github/configuring-notifications#automatic-watching)”。
-To see an overview of your repository subscriptions, see "[Reviewing repositories that you're watching](#reviewing-repositories-that-youre-watching)." Many people forget about repositories that they've chosen to watch in the past. From the "Watched repositories" page you can quickly unwatch repositories. For more information on ways to unsubscribe, see "[Managing subscriptions](/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions)." 许多人忘记了他们过去选择关注的仓库。 从“Watched repositories(已关注仓库)”页面,您可以快速取消关注仓库。 有关取消订阅方式的更多信息,请参阅“[管理订阅](/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions)”。
+To see an overview of your repository subscriptions, see "[Reviewing repositories that you're watching](#reviewing-repositories-that-youre-watching)." Many people forget about repositories that they've chosen to watch in the past. From the "Watched repositories" page you can quickly unwatch repositories. For more information on ways to unsubscribe, see "[Managing subscriptions](/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions)."
+{% if currentVersion == "free-pro-team@latest" %}
+{% tip %}
+
+**Tip:** You can select the types of event to be notified of by using the **Custom** option of the **Watch/Unwatch** dropdown list in your [watching page](https://github.com/watching) or on any repository page on {% data variables.product.prodname_dotcom_the_website %}. For more information, see "[Configuring your watch settings for an individual repository](#configuring-your-watch-settings-for-an-individual-repository)" below.
+
+{% endtip %}
+{% endif %}
+
+许多人忘记了他们过去选择关注的仓库。 从“Watched repositories(已关注仓库)”页面,您可以快速取消关注仓库。 For more information on ways to unsubscribe, see "[Unwatch recommendations](https://github.blog/changelog/2020-11-10-unwatch-recommendations/)" on {% data variables.product.prodname_blog %} and "[Managing your subscriptions](/github/managing-subscriptions-and-notifications-on-github/managing-your-subscriptions)." You can also create a triage worflow to help with the notifications you receive. For guidance on triage workflows, see "[Customizing a workflow for triaging your notifications](/github/managing-subscriptions-and-notifications-on-github/customizing-a-workflow-for-triaging-your-notifications)."
### 查看所有订阅
@@ -55,20 +64,38 @@ To see an overview of your repository subscriptions, see "[Reviewing repositorie
### 查看您目前关注的仓库
1. 在左侧边栏中的仓库列表下,使用“Manage notifications(管理通知)”下拉菜单单击 **Watched repositories(已关注的仓库)**。 
-
-3. 评估您正在关注的仓库,确定它们更新是否仍然相关和有用。 关注某仓库后,您将收到该仓库所有对话的通知。
-
+2. 评估您正在关注的仓库,确定它们更新是否仍然相关和有用。 关注某仓库后,您将收到该仓库所有对话的通知。
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}

+{% endif %}
+{% if currentVersion == "free-pro-team@latest" %}
+ 
+{% endif %}
{% tip %}
- **提示:**不关注仓库,只考虑关注仓库的发行版,或者完全取消关注仓库。 取消关注仓库后,当您被@提及或参与帖子时仍然会收到通知。 只关注仓库的发行版时,仅当有新版本发行、您参与帖子或者您或您所在团队被@提及时才会收到通知。
+ **Tip:** Instead of watching a repository, consider only receiving notifications {% if currentVersion == "free-pro-team@latest" %}when there are updates to issues, pull requests, releases or discussions (if enabled for the repository), or any combination of these options,{% else %}for releases in a repository,{% endif %} or completely unwatching a repository.
+
+ 取消关注仓库后,当您被@提及或参与帖子时仍然会收到通知。 When you configure to receive notifications for certain event types, you're only notified when there are updates to these event types in the repository, you're participating in a thread, or you or a team you're on is @mentioned.
{% endtip %}
### 配置单个仓库的关注设置
-您可以选择关注还是取消关注单个仓库。 也可以选择仅在有新发行版时才收到通知或者忽略单个仓库。
+您可以选择关注还是取消关注单个仓库。 You can also choose to only be notified of {% if currentVersion == "free-pro-team@latest" %}certain event types such as issues, pull requests, discussions (if enabled for the repository) and {% endif %}new releases, or completely ignore an individual repository.
{% data reusables.repositories.navigate-to-repo %}
-2. 在右上角,单击“Watch(关注)”下拉菜单选择关注选项。 
+2. 在右上角,单击“Watch(关注)”下拉菜单选择关注选项。
+{% if currentVersion == "github-ae@latest" or currentVersion ver_gt "enterprise-server@2.20" %}
+ 
+{% endif %}
+{% if currentVersion == "free-pro-team@latest" %}
+ 
+{% data reusables.notifications-v2.custom-notifications-beta %}
+The **Custom** option allows you to further customize notifications so that you're only notified when specific events happen in the repository, in addition to participating and @mentions.
+
+ 
+
+If you select "Issues", you will be notified about, and subscribed to, updates on every issue (including those that existed prior to you selecting this option) in the repository. If you're @mentioned in a pull request in this repository, you'll receive notifications for that too, and you'll be subscribed to updates on that specific pull request, in addition to being notified about issues.
+
+{% endif %}
diff --git a/translations/zh-CN/content/github/managing-your-work-on-github/creating-an-issue.md b/translations/zh-CN/content/github/managing-your-work-on-github/creating-an-issue.md
index 84fd2c223e7f..3e06c4df51a2 100644
--- a/translations/zh-CN/content/github/managing-your-work-on-github/creating-an-issue.md
+++ b/translations/zh-CN/content/github/managing-your-work-on-github/creating-an-issue.md
@@ -1,6 +1,7 @@
---
title: 创建议题
intro: '议题可用于跟踪漏洞、增强功能或其他请求。'
+permissions: 'People with read permissions can create an issue in a repository where issues are enabled.'
redirect_from:
- /articles/creating-an-issue
versions:
@@ -9,8 +10,6 @@ versions:
github-ae: '*'
---
-{% data reusables.repositories.create-issue-in-public-repository %}
-
您可以根据现有拉取请求中的代码打开新议题。 更多信息请参阅“[从代码打开议题](/github/managing-your-work-on-github/opening-an-issue-from-code)”。
可以直接从议题或拉取请求审查中的评论打开新议题。 更多信息请参阅“[从评论打开议题](/github/managing-your-work-on-github/opening-an-issue-from-a-comment)”。
diff --git a/translations/zh-CN/content/github/managing-your-work-on-github/linking-a-pull-request-to-an-issue.md b/translations/zh-CN/content/github/managing-your-work-on-github/linking-a-pull-request-to-an-issue.md
index 86547a3c9a34..40b9445af274 100644
--- a/translations/zh-CN/content/github/managing-your-work-on-github/linking-a-pull-request-to-an-issue.md
+++ b/translations/zh-CN/content/github/managing-your-work-on-github/linking-a-pull-request-to-an-issue.md
@@ -50,7 +50,7 @@ You can link a pull request to an issue by using a supported keyword in the pull
* fix
* fixes
* fixed
-* 解决
+* resolve
* resolves
* resolved
diff --git a/translations/zh-CN/content/github/managing-your-work-on-github/opening-an-issue-from-a-comment.md b/translations/zh-CN/content/github/managing-your-work-on-github/opening-an-issue-from-a-comment.md
index 370acb026843..828cf2b2dbb5 100644
--- a/translations/zh-CN/content/github/managing-your-work-on-github/opening-an-issue-from-a-comment.md
+++ b/translations/zh-CN/content/github/managing-your-work-on-github/opening-an-issue-from-a-comment.md
@@ -1,6 +1,7 @@
---
title: 从评论打开议题
intro: 您可以从议题或拉取请求中的特定评论行打开新议题。
+permissions: 'People with read permissions can create an issue in a repository where issues are enabled.'
versions:
free-pro-team: '*'
enterprise-server: '*'
@@ -9,6 +10,8 @@ versions:
从评论打开议题时,该议题包含一个代码段,显示评论的原始发布位置。
+{% data reusables.repositories.administrators-can-disable-issues %}
+
1. 导航到您要打开其评论的议题。
2. 在该评论中,单击 {% octicon "kebab-horizontal" aria-label="The horizontal kebab icon" %}。 
diff --git a/translations/zh-CN/content/github/managing-your-work-on-github/opening-an-issue-from-code.md b/translations/zh-CN/content/github/managing-your-work-on-github/opening-an-issue-from-code.md
index e96efa75f57a..c8cd2a0f86bc 100644
--- a/translations/zh-CN/content/github/managing-your-work-on-github/opening-an-issue-from-code.md
+++ b/translations/zh-CN/content/github/managing-your-work-on-github/opening-an-issue-from-code.md
@@ -1,6 +1,7 @@
---
title: 从代码打开议题
intro: 您可以从文件或拉取请求的特定代码行打开新议题。
+permissions: 'People with read permissions can create an issue in a repository where issues are enabled.'
redirect_from:
- /articles/opening-an-issue-from-code
versions:
@@ -13,7 +14,7 @@ versions:

-{% data reusables.repositories.create-issue-in-public-repository %}
+{% data reusables.repositories.administrators-can-disable-issues %}
{% data reusables.repositories.navigate-to-repo %}
2. 找到要在议题中引用的代码:
diff --git a/translations/zh-CN/content/github/managing-your-work-on-github/transferring-an-issue-to-another-repository.md b/translations/zh-CN/content/github/managing-your-work-on-github/transferring-an-issue-to-another-repository.md
index 12d40eafa2ee..e96b2c3fef6d 100644
--- a/translations/zh-CN/content/github/managing-your-work-on-github/transferring-an-issue-to-another-repository.md
+++ b/translations/zh-CN/content/github/managing-your-work-on-github/transferring-an-issue-to-another-repository.md
@@ -11,7 +11,7 @@ versions:
要将打开的议题转让给另一个仓库,必须对议题所在的仓库以及议题要转让到的仓库都有写入权限。 更多信息请参阅“[组织的仓库权限级别](/articles/repository-permission-levels-for-an-organization)”。
-您只能在同一用户或组织帐户拥有的仓库之间转让议题。 您无法将私有仓库的议题转让给公共仓库。
+You can only transfer issues between repositories owned by the same user or organization account.{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}You can't transfer an issue from a private repository to a public repository.{% endif %}
转让议题时,评论和受理人将保留。 The issue's labels and milestones are not retained. This issue will stay on any user-owned or organization-wide project boards and be removed from any repository project boards. 更多信息请参阅“[关于项目板](/articles/about-project-boards)”。
diff --git a/translations/zh-CN/content/github/searching-for-information-on-github/about-searching-on-github.md b/translations/zh-CN/content/github/searching-for-information-on-github/about-searching-on-github.md
index c23a63f9ee25..eaf6392f0739 100644
--- a/translations/zh-CN/content/github/searching-for-information-on-github/about-searching-on-github.md
+++ b/translations/zh-CN/content/github/searching-for-information-on-github/about-searching-on-github.md
@@ -14,7 +14,7 @@ versions:
github-ae: '*'
---
-您可以全局搜索所有 {% data variables.product.product_name %},也可搜索特定仓库或组织。
+{% data reusables.search.you-can-search-globally %}
- 要全局搜索所有 {% data variables.product.product_name %},请在页面顶部的搜索字段中输入您要查找的内容,然后在搜索下拉菜单中选择“所有{% data variables.product.prodname_dotcom %}”。
- 要在特定仓库或组织中搜索,请导航到该仓库或组织页面,在页面顶部的搜索字段中输入要查找的内容,然后按 **Enter**。
@@ -23,7 +23,8 @@ versions:
**注意:**
-- {% data reusables.search.required_login %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- {% data reusables.search.required_login %}{% endif %}
- {% data variables.product.prodname_pages %} 网站在 {% data variables.product.product_name %} 上不可搜索。 但如果源代码内容存在于仓库的默认分支中,您可以使用代码搜索来搜索。 更多信息请参阅“[搜索代码](/articles/searching-code)”。 有关 {% data variables.product.prodname_pages %} 的更多信息,请参阅“[什么是 GitHub Pages? ](/articles/what-is-github-pages/)”
- Currently our search doesn't support exact matching.
- Whenever you are searching in code files, only the first two results in each file will be returned.
@@ -36,7 +37,7 @@ versions:
### {% data variables.product.prodname_dotcom %} 上的搜索类型
-您可以在所有公共 {% data variables.product.product_name %} 仓库以及您可以访问的所有 {% data variables.product.product_name %} 仓库中搜索以下类型的信息:
+You can search for the following information across all repositories you can access on {% data variables.product.product_location %}.
- [仓库](/articles/searching-for-repositories)
- [主题](/articles/searching-topics)
diff --git a/translations/zh-CN/content/github/searching-for-information-on-github/searching-code.md b/translations/zh-CN/content/github/searching-for-information-on-github/searching-code.md
index ab8e67b7f7c0..c67da3691eee 100644
--- a/translations/zh-CN/content/github/searching-for-information-on-github/searching-code.md
+++ b/translations/zh-CN/content/github/searching-for-information-on-github/searching-code.md
@@ -11,7 +11,7 @@ versions:
github-ae: '*'
---
-您可以在所有 {% data variables.product.product_name %} 内全局搜索代码,也可以在特定仓库或组织内搜索代码。 要在所有公共仓库内搜索代码,您必须登录到 {% data variables.product.product_name %} 帐户。 更多信息请参阅“[关于在 GitHub 上搜索](/articles/about-searching-on-github)”。
+{% data reusables.search.you-can-search-globally %} For more information, see "[About searching on GitHub](/articles/about-searching-on-github)."
您只能使用这些代码搜索限定符搜索代码。 搜索代码时,专用于仓库、用户或提交的搜索限定符将不起作用。
@@ -21,13 +21,13 @@ versions:
由于搜索代码的复杂性,执行搜索的方式有一些限制:
-- {% data reusables.search.required_login %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- {% data reusables.search.required_login %}{% endif %}
- [复刻](/articles/about-forks)中的代码仅当复刻的星号超过父级仓库时可搜索。 星号少于父仓库的复刻**不**为代码搜索编索引。 要在搜索结果中包括星号比其父项多的复刻,您需要将 `fork:true` 或 `fork:only` 添加到查询。 更多信息请参阅“[在复刻中搜索](/articles/searching-in-forks)”。
- Only the _default branch_ is indexed for code search.{% if currentVersion == "free-pro-team@latest" %}
- 只有小于 384 KB 的文件可搜索。{% else %}* 只有小于 5 MB 的文件可搜索。
- 只有每个文件的前 500 KB 可搜索。{% endif %}
- 只有少于 500,000 个文件的仓库可搜索。
-- 登录的用户可以搜索所有公共仓库。
- 除了 [`filename`](#search-by-filename) 搜索以外,搜索源代码时必须始终包括至少一个搜索词。 例如,搜索 [`language:javascript`](https://github.com/search?utf8=%E2%9C%93&q=language%3Ajavascript&type=Code&ref=searchresults) 无效,而搜索 [`amazing language:javascript`](https://github.com/search?utf8=%E2%9C%93&q=amazing+language%3Ajavascript&type=Code&ref=searchresults) 有效。
- 搜索结果最多可显示同一文件的两个分段,但文件内可能有更多结果。
- 您无法使用以下通配符作为搜索查询的一部分:. , : ; / \ ` ' " = * ! ? # $ & + ^ | ~ < > ( ) { } [ ]
. 搜索只会忽略这些符号。
diff --git a/translations/zh-CN/content/github/searching-for-information-on-github/searching-commits.md b/translations/zh-CN/content/github/searching-for-information-on-github/searching-commits.md
index 626ffc3e9fc7..444f6c68a07a 100644
--- a/translations/zh-CN/content/github/searching-for-information-on-github/searching-commits.md
+++ b/translations/zh-CN/content/github/searching-for-information-on-github/searching-commits.md
@@ -96,14 +96,11 @@ versions:
| org:ORGNAME
| [**test org:github**](https://github.com/search?utf8=%E2%9C%93&q=test+org%3Agithub&type=Commits) 匹配 @github 拥有的仓库中含有 "test" 字样的提交消息。 |
| repo:USERNAME/REPO
| [**language repo:defunkt/gibberish**](https://github.com/search?utf8=%E2%9C%93&q=language+repo%3Adefunkt%2Fgibberish&type=Commits) 匹配 @defunkt 的 "gibberish" 仓库中含有 "language" 字样的提交消息。 |
-### 过滤公共或私有仓库
+### Filter by repository visibility
-`is` 限定符匹配公共或私有提交。
+The `is` qualifier matches commits from repositories with the specified visibility. For more information, see "[About repository visibility](/github/creating-cloning-and-archiving-repositories/about-repository-visibility).
-| 限定符 | 示例 |
-| ------------ | ------------------------------------------------------------------------------- |
-| `is:public` | [**is:public**](https://github.com/search?q=is%3Apublic&type=Commits) 匹配公共提交。 |
-| `is:private` | [**is:private**](https://github.com/search?q=is%3Aprivate&type=Commits) 匹配私有提交。 |
+| Qualifier | Example | ------------- | ------------- |{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %} | `is:public` | [**is:public**](https://github.com/search?q=is%3Apublic&type=Commits) matches commits to public repositories.{% endif %} | `is:internal` | [**is:internal**](https://github.com/search?q=is%3Ainternal&type=Commits) matches commits to internal repositories. | `is:private` | [**is:private**](https://github.com/search?q=is%3Aprivate&type=Commits) matches commits to private repositories.
### 延伸阅读
diff --git a/translations/zh-CN/content/github/searching-for-information-on-github/searching-for-repositories.md b/translations/zh-CN/content/github/searching-for-information-on-github/searching-for-repositories.md
index d86b184dde44..ad58a1c41b9f 100644
--- a/translations/zh-CN/content/github/searching-for-information-on-github/searching-for-repositories.md
+++ b/translations/zh-CN/content/github/searching-for-information-on-github/searching-for-repositories.md
@@ -10,7 +10,7 @@ versions:
github-ae: '*'
---
-您可以在所有 {% data variables.product.product_name %} 内全局搜索仓库,也可以在特定组织内搜索仓库。 更多信息请参阅“[关于在 {% data variables.product.prodname_dotcom %} 上搜索](/articles/about-searching-on-github)”。
+您可以在所有 {% data variables.product.product_location %} 内全局搜索仓库,也可以在特定组织内搜索仓库。 更多信息请参阅“[关于在 {% data variables.product.prodname_dotcom %} 上搜索](/articles/about-searching-on-github)”。
要在搜索结果中包括复刻,您需要将 `fork:true` 或 `fork:only` 添加到查询。 更多信息请参阅“[在复刻中搜索](/articles/searching-in-forks)”。
@@ -20,22 +20,22 @@ versions:
通过 `in` 限定符,您可以将搜索限制为仓库名称、仓库说明、自述文件内容或这些的任意组合。 如果省略此限定符,则只搜索仓库名称和说明。
-| 限定符 | 示例 |
-| ----------------- | ---------------------------------------------------------------------------------------------------------------------------------------- |
-| `in:name` | [**jquery in:name**](https://github.com/search?q=jquery+in%3Aname&type=Repositories) 匹配其名称中含有 "jquery" 的仓库。 |
-| `in:description` | [**jquery in:name,description**](https://github.com/search?q=jquery+in%3Aname%2Cdescription&type=Repositories) 匹配其名称或说明中含有 "jquery" 的仓库。 |
-| `in:readme` | [**jquery in:readme**](https://github.com/search?q=jquery+in%3Areadme&type=Repositories) 匹配其自述文件中提及 "jquery" 的仓库。 |
-| `repo:owner/name` | [**repo:octocat/hello-world**](https://github.com/search?q=repo%3Aoctocat%2Fhello-world) 匹配特定仓库名称。 |
+| 限定符 | 示例 |
+| ----------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `in:name` | [**jquery in:name**](https://github.com/search?q=jquery+in%3Aname&type=Repositories) matches repositories with "jquery" in the repository name. |
+| `in:description` | [**jquery in:name,description**](https://github.com/search?q=jquery+in%3Aname%2Cdescription&type=Repositories) matches repositories with "jquery" in the repository name or description. |
+| `in:readme` | [**jquery in:readme**](https://github.com/search?q=jquery+in%3Areadme&type=Repositories) matches repositories mentioning "jquery" in the repository's README file. |
+| `repo:owner/name` | [**repo:octocat/hello-world**](https://github.com/search?q=repo%3Aoctocat%2Fhello-world) 匹配特定仓库名称。 |
### 基于仓库的内容搜索
-您可以使用 `in:readme` 限定符,通过搜索其自述文件中的内容来查找仓库。
+You can find a repository by searching for content in the repository's README file using the `in:readme` qualifier. 更多信息请参阅“[关于自述文件](/github/creating-cloning-and-archiving-repositories/about-readmes)”。
除了使用 `in:readme` 以外,无法通过搜索仓库内的特定内容来查找仓库。 要搜索仓库内的特定文件或内容,您可以使用查找器或代码特定的搜索限定符。 更多信息请参阅“[在 {% data variables.product.prodname_dotcom %} 上查找文件](/articles/finding-files-on-github)”和“[搜索代码](/articles/searching-code)”。
-| 限定符 | 示例 |
-| ----------- | -------------------------------------------------------------------------------------------------------------------- |
-| `in:readme` | [**octocat in:readme**](https://github.com/search?q=octocat+in%3Areadme&type=Repositories) 匹配其自述文件中提及 "octocat" 的仓库。 |
+| 限定符 | 示例 |
+| ----------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `in:readme` | [**octocat in:readme**](https://github.com/search?q=octocat+in%3Areadme&type=Repositories) matches repositories mentioning "octocat" in the repository's README file. |
### 在用户或组织的仓库内搜索
@@ -48,7 +48,7 @@ versions:
### 按仓库大小搜索
-`size` 限定符使用[大于、小于和范围限定符](/articles/understanding-the-search-syntax)查找匹配特定大小(以千字节为单位)的仓库。
+The `size` qualifier finds repositories that match a certain size (in kilobytes), using greater than, less than, and range qualifiers. For more information, see "[Understanding the search syntax](/github/searching-for-information-on-github/understanding-the-search-syntax)."
| 限定符 | 示例 |
| ------------------------- | -------------------------------------------------------------------------------------------------------------- |
@@ -59,7 +59,7 @@ versions:
### 按关注者数量搜索
-您可以使用 `followers` 限定符以及[大于、小于和范围限定符](/articles/understanding-the-search-syntax)基于仓库拥有的关注者数量过滤仓库。
+You can filter repositories based on the number of users who follow the repositories, using the `followers` qualifier with greater than, less than, and range qualifiers. For more information, see "[Understanding the search syntax](/github/searching-for-information-on-github/understanding-the-search-syntax)."
| 限定符 | 示例 |
| ------------------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -68,7 +68,7 @@ versions:
### 按复刻数量搜索
-`forks` 限定符使用[大于、小于和范围限定符](/articles/understanding-the-search-syntax)指定仓库应具有的复刻数量。
+The `forks` qualifier specifies the number of forks a repository should have, using greater than, less than, and range qualifiers. For more information, see "[Understanding the search syntax](/github/searching-for-information-on-github/understanding-the-search-syntax)."
| 限定符 | 示例 |
| ------------------------- | -------------------------------------------------------------------------------------------------------------- |
@@ -79,7 +79,7 @@ versions:
### 按星号数量搜索
-您可以使用[大于、小于和范围限定符](/articles/understanding-the-search-syntax)基于仓库具有的[星标](/articles/saving-repositories-with-stars)数量搜索仓库
+You can search repositories based on the number of stars the repositories have, using greater than, less than, and range qualifiers. For more information, see "[Saving repositories with stars](/github/getting-started-with-github/saving-repositories-with-stars)" and "[Understanding the search syntax](/github/searching-for-information-on-github/understanding-the-search-syntax)."
| 限定符 | 示例 |
| ------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -103,7 +103,7 @@ versions:
### 按语言搜索
-您可以基于其编写采用的主要语言搜索仓库。
+You can search repositories based on the language of the code in the repositories.
| 限定符 | 示例 |
| ------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------------- |
@@ -111,7 +111,7 @@ versions:
### 按主题搜索
-您可以查找归类为特定[主题](/articles/classifying-your-repository-with-topics)的所有仓库。
+You can find all of the repositories that are classified with a particular topic. 更多信息请参阅“[使用主题对仓库分类](/github/administering-a-repository/classifying-your-repository-with-topics)”。
| 限定符 | 示例 |
| ------------------------- | --------------------------------------------------------------------------------------------------------------------------------------- |
@@ -119,53 +119,55 @@ versions:
### 按主题数量搜索
-您可以使用 `topics` 限定符以及[大于、小于和范围限定符](/articles/understanding-the-search-syntax)按应用于仓库的[主题](/articles/classifying-your-repository-with-topics)数量搜索仓库。
+You can search repositories by the number of topics that have been applied to the repositories, using the `topics` qualifier along with greater than, less than, and range qualifiers. For more information, see "[Classifying your repository with topics](/github/administering-a-repository/classifying-your-repository-with-topics)" and "[Understanding the search syntax](/github/searching-for-information-on-github/understanding-the-search-syntax)."
| 限定符 | 示例 |
| -------------------------- | -------------------------------------------------------------------------------------------------------------------------- |
| topics:n
| [**topics:5**](https://github.com/search?utf8=%E2%9C%93&q=topics%3A5&type=Repositories&ref=searchresults) 匹配具有五个主题的仓库。 |
| | [**topics:>3**](https://github.com/search?utf8=%E2%9C%93&q=topics%3A%3E3&type=Repositories&ref=searchresults) 匹配超过三个主题的仓库。 |
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
### 按许可搜索
-您可以按其[许可](/articles/licensing-a-repository)搜索仓库。 您必须使用[许可关键词](/articles/licensing-a-repository/#searching-github-by-license-type)按特定许可或许可系列过滤仓库。
+You can search repositories by the type of license in the repositories. You must use a license keyword to filter repositories by a particular license or license family. 更多信息请参阅“[许可仓库](/github/creating-cloning-and-archiving-repositories/licensing-a-repository)”。
| 限定符 | 示例 |
| -------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------ |
| license:LICENSE_KEYWORD
| [**license:apache-2.0**](https://github.com/search?utf8=%E2%9C%93&q=license%3Aapache-2.0&type=Repositories&ref=searchresults) 匹配根据 Apache License 2.0 授权的仓库。 |
-### 按公共或私有仓库搜索
+{% endif %}
+
+### Search by repository visibility
-您可以基于仓库是公共还是私有来过滤搜索。
+You can filter your search based on the visibility of the repositories. 更多信息请参阅“[关于仓库可见性](/github/creating-cloning-and-archiving-repositories/about-repository-visibility)”。
-| 限定符 | 示例 |
-| ------------ | ------------------------------------------------------------------------------------------------------------------------------------ |
-| `is:public` | [**is:public org:github**](https://github.com/search?q=is%3Apublic+org%3Agithub&type=Repositories&utf8=%E2%9C%93) 匹配 GitHub 拥有的公共仓库。 |
-| `is:private` | [**is:private pages**](https://github.com/search?utf8=%E2%9C%93&q=pages+is%3Aprivate&type=Repositories) 匹配您有访问权限且包含 "pages" 字样的私有仓库。 |
+| Qualifier | Example | ------------- | ------------- |{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %} | `is:public` | [**is:public org:github**](https://github.com/search?q=is%3Apublic+org%3Agithub&type=Repositories) matches public repositories owned by {% data variables.product.company_short %}.{% endif %} | `is:internal` | [**is:internal test**](https://github.com/search?q=is%3Ainternal+test&type=Repositories) matches internal repositories that you can access and contain the word "test". | `is:private` | [**is:private pages**](https://github.com/search?q=is%3Aprivate+pages&type=Repositories) matches private repositories that you can access and contain the word "pages."
{% if currentVersion == "free-pro-team@latest" %}
### 基于仓库是否为镜像搜索
-您可以根据仓库是否为镜像以及托管于其他位置托管来搜索它们。 更多信息请参阅“[寻找在 {% data variables.product.prodname_dotcom %} 上参与开源项目的方法](/github/getting-started-with-github/finding-ways-to-contribute-to-open-source-on-github)”。
+You can search repositories based on whether the repositories are mirrors and hosted elsewhere. 更多信息请参阅“[寻找在 {% data variables.product.prodname_dotcom %} 上参与开源项目的方法](/github/getting-started-with-github/finding-ways-to-contribute-to-open-source-on-github)”。
-| 限定符 | 示例 |
-| -------------- | ------------------------------------------------------------------------------------------------------------------------ |
-| `mirror:true` | [**mirror:true GNOME**](https://github.com/search?utf8=%E2%9C%93&q=mirror%3Atrue+GNOME&type=) 匹配是镜像且包含 "GNOME" 字样的仓库。 |
-| `mirror:false` | [**mirror:false GNOME**](https://github.com/search?utf8=%E2%9C%93&q=mirror%3Afalse+GNOME&type=) 匹配并非镜像且包含 "GNOME" 字样的仓库。 |
+| 限定符 | 示例 |
+| -------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `mirror:true` | [**mirror:true GNOME**](https://github.com/search?utf8=%E2%9C%93&q=mirror%3Atrue+GNOME&type=) matches repositories that are mirrors and contain the word "GNOME." |
+| `mirror:false` | [**mirror:false GNOME**](https://github.com/search?utf8=%E2%9C%93&q=mirror%3Afalse+GNOME&type=) matches repositories that are not mirrors and contain the word "GNOME." |
{% endif %}
### 基于仓库是否已存档搜索
-您可以基于仓库是否[已存档](/articles/about-archiving-repositories)来搜索仓库。
+You can search repositories based on whether or not the repositories are archived. For more information, see "[About archiving repositories](/github/creating-cloning-and-archiving-repositories/about-archiving-repositories)."
-| 限定符 | 示例 |
-| ---------------- | --------------------------------------------------------------------------------------------------------------------------- |
-| `archived:true` | [**archived:true GNOME**](https://github.com/search?utf8=%E2%9C%93&q=archived%3Atrue+GNOME&type=) 匹配已存档且包含 "GNOME" 字样的仓库。 |
-| `archived:false` | [**archived:false GNOME**](https://github.com/search?utf8=%E2%9C%93&q=archived%3Afalse+GNOME&type=) 匹配未存档且包含 "GNOME" 字样的仓库。 |
+| 限定符 | 示例 |
+| ---------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| `archived:true` | [**archived:true GNOME**](https://github.com/search?utf8=%E2%9C%93&q=archived%3Atrue+GNOME&type=) matches repositories that are archived and contain the word "GNOME." |
+| `archived:false` | [**archived:false GNOME**](https://github.com/search?utf8=%E2%9C%93&q=archived%3Afalse+GNOME&type=) matches repositories that are not archived and contain the word "GNOME." |
{% if currentVersion == "free-pro-team@latest" %}
+
### 基于具有 `good first issue` 或 `help wanted` 标签的议题数量搜索
您可以使用限定符 `help-wanted-issues:>n` 和 `good-first-issues:>n` 搜索具有最少数量标签为 `help-wanted` 或 `good-first-issue` 议题的仓库。 更多信息请参阅“[通过标签鼓励对项目做出有益的贡献](/github/building-a-strong-community/encouraging-helpful-contributions-to-your-project-with-labels)”。
@@ -174,6 +176,7 @@ versions:
| -------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| `good-first-issues:>n` | [**good-first-issues:>2 javascript**](https://github.com/search?utf8=%E2%9C%93&q=javascript+good-first-issues%3A%3E2&type=) 匹配具有超过两个标签为 `good-first-issue` 的议题且包含 "javascript" 字样的仓库。 |
| `help-wanted-issues:>n` | [**help-wanted-issues:>4 react**](https://github.com/search?utf8=%E2%9C%93&q=react+help-wanted-issues%3A%3E4&type=) 匹配具有超过四个标签为 `help-wanted` 的议题且包含 "React" 字样的仓库。 |
+
{% endif %}
### 延伸阅读
diff --git a/translations/zh-CN/content/github/searching-for-information-on-github/searching-issues-and-pull-requests.md b/translations/zh-CN/content/github/searching-for-information-on-github/searching-issues-and-pull-requests.md
index ba9fcbe83c65..91f818b77b7a 100644
--- a/translations/zh-CN/content/github/searching-for-information-on-github/searching-issues-and-pull-requests.md
+++ b/translations/zh-CN/content/github/searching-for-information-on-github/searching-issues-and-pull-requests.md
@@ -64,66 +64,63 @@ versions:
| `is:open` | [**performance is:open is:issue**](https://github.com/search?q=performance+is%3Aopen+is%3Aissue&type=Issues) 匹配含有 "performance" 字样的开放议题。 |
| `is:closed` | [**android is:closed**](https://github.com/search?utf8=%E2%9C%93&q=android+is%3Aclosed&type=) 匹配含有 "android" 字样的已关闭议题和拉取请求。 |
-### 按公共或私有仓库搜索
+### Filter by repository visibility
-如果您[在所有 {% data variables.product.product_name %} 内搜索](https://github.com/search),基于仓库是公共还是私有来过滤结果可能非常有用。 您可以使用 `is:public` 和 `is:private` 实现此操作。
+You can filter by the visibility of the repository containing the issues and pull requests using the `is` qualifier. 更多信息请参阅“[关于仓库可见性](/github/creating-cloning-and-archiving-repositories/about-repository-visibility)”。
-| 限定符 | 示例 |
-| ------------ | ---------------------------------------------------------------------------------------------------------------------- |
-| `is:public` | [**is:public**](https://github.com/search?q=is%3Apublic&type=Issues) 匹配所有公共仓库中的议题和拉取请求。 |
-| `is:private` | [**is:private cupcake**](https://github.com/search?q=is%3Aprivate&type=Issues) 匹配您具有访问权限的私有仓库中含有 "cupcake" 字样的议题和拉取请求。 |
+| Qualifier | Example | ------------- | ------------- |{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %} | `is:public` | [**is:public**](https://github.com/search?q=is%3Apublic&type=Issues) matches issues and pull requests in public repositories.{% endif %}{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.20" or currentVersion == "github-ae@latest" %} | `is:internal` | [**is:internal**](https://github.com/search?q=is%3Ainternal&type=Issues) matches issues and pull requests in internal repositories.{% endif %} | `is:private` | [**is:private cupcake**](https://github.com/search?q=is%3Aprivate+cupcake&type=Issues) matches issues and pull requests that contain the word "cupcake" in private repositories you can access.
### 按作者搜索
`author` 限定符查找由特定用户或集成帐户创建的议题和拉取请求。
-| 限定符 | 示例 |
-| ------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------- |
-| author:USERNAME
| [**cool author:gjtorikian**](https://github.com/search?q=cool+author%3Agjtorikian&type=Issues) 匹配含有 "cool" 字样、由 @gjtorikian 创建的议题和拉取请求。 |
-| | [**bootstrap in:body author:mdo**](https://github.com/search?q=bootstrap+in%3Abody+author%3Amdo&type=Issues) 匹配由 @mdo 撰写、正文中含有 "bootstrap" 字样的议题。 |
-| author:app/USERNAME
| [**author:app/robot**](https://github.com/search?q=author%3Aapp%2Frobot&type=Issues) 匹配由名为 "robot" 的集成帐户创建的议题。 |
+| 限定符 | 示例 |
+| ------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| author:USERNAME
| [**cool author:gjtorikian**](https://github.com/search?q=cool+author%3Agjtorikian&type=Issues) matches issues and pull requests with the word "cool" that were created by @gjtorikian. |
+| | [**bootstrap in:body author:mdo**](https://github.com/search?q=bootstrap+in%3Abody+author%3Amdo&type=Issues) matches issues written by @mdo that contain the word "bootstrap" in the body. |
+| author:app/USERNAME
| [**author:app/robot**](https://github.com/search?q=author%3Aapp%2Frobot&type=Issues) matches issues created by the integration account named "robot." |
### 按受理人搜索
-`assignee` 限定符查找分配给特定用户的议题和拉取请求。 您无法搜索具有 _any_ 受理人的议题和拉取请求,但可以搜索[没有受理人的议题和拉取请求](#search-by-missing-metadata)。
+`assignee` 限定符查找分配给特定用户的议题和拉取请求。 You cannot search for issues and pull requests that have _any_ assignee, however, you can search for [issues and pull requests that have no assignee](#search-by-missing-metadata).
-| 限定符 | 示例 |
-| ------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| assignee:USERNAME
| [**assignee:vmg repo:libgit2/libgit2**](https://github.com/search?utf8=%E2%9C%93&q=assignee%3Avmg+repo%3Alibgit2%2Flibgit2&type=Issues) 匹配分配给 @vmg 的 libgit2 项目 libgit2 中的议题和拉取请求。 |
+| 限定符 | 示例 |
+| ------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| assignee:USERNAME
| [**assignee:vmg repo:libgit2/libgit2**](https://github.com/search?utf8=%E2%9C%93&q=assignee%3Avmg+repo%3Alibgit2%2Flibgit2&type=Issues) matches issues and pull requests in libgit2's project libgit2 that are assigned to @vmg. |
### 按提及搜索
`mentions` 限定符查找提及特定用户的议题。 更多信息请参阅“[提及人员和团队](/articles/basic-writing-and-formatting-syntax/#mentioning-people-and-teams)”。
-| 限定符 | 示例 |
-| ------------------------- | ---------------------------------------------------------------------------------------------------------------------------------- |
-| mentions:USERNAME
| [**resque mentions:defunkt**](https://github.com/search?q=resque+mentions%3Adefunkt&type=Issues) 匹配含有 "resque" 字样、提及 @defunkt 的议题。 |
+| 限定符 | 示例 |
+| ------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| mentions:USERNAME
| [**resque mentions:defunkt**](https://github.com/search?q=resque+mentions%3Adefunkt&type=Issues) matches issues with the word "resque" that mention @defunkt. |
### 按团队提及搜索
对于您所属的组织和团队,您可以使用 `team` 限定符查找提及该组织内特定团队的议题或拉取请求。 将这些示例名称替换为您的组织和团队的名称以执行搜索。
-| 限定符 | 示例 |
-| ------------------------- | ------------------------------------------------------------- |
-| team:ORGNAME/TEAMNAME
| **team:jekyll/owners** 匹配提及 `@jekyll/owners` 团队的议题。 |
-| | **team:myorg/ops is:open is:pr** 匹配提及 `@myorg/ops` 团队的打开拉取请求。 |
+| 限定符 | 示例 |
+| ------------------------- | ----------------------------------------------------------------------------------------------------- |
+| team:ORGNAME/TEAMNAME
| **team:jekyll/owners** matches issues where the `@jekyll/owners` team is mentioned. |
+| | **team:myorg/ops is:open is:pr** matches open pull requests where the `@myorg/ops` team is mentioned. |
### 按评论者搜索
`commenter` 限定符查找含有来自特定用户评论的议题。
-| 限定符 | 示例 |
-| ------------------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| commenter:USERNAME
| [**github commenter:defunkt org:github**](https://github.com/search?utf8=%E2%9C%93&q=github+commenter%3Adefunkt+org%3Agithub&type=Issues) 匹配位于 GitHub 拥有的仓库中、含有 "github" 字样且由 @defunkt 评论的议题。 |
+| 限定符 | 示例 |
+| ------------------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| commenter:USERNAME
| [**github commenter:defunkt org:github**](https://github.com/search?utf8=%E2%9C%93&q=github+commenter%3Adefunkt+org%3Agithub&type=Issues) matches issues in repositories owned by GitHub, that contain the word "github," and have a comment by @defunkt. |
### 按议题或拉取请求中涉及的用户搜索
您可以使用 `involves` 限定符查找以某种方式涉及特定用户的议题。 `involves` 限定符是单一用户 `author`、`assignee`、`mentions` 和 `commenter` 限定符之间的逻辑 OR(或)。 换句话说,此限定符查找由特定用户创建、分配给该用户、提及该用户或由该用户评论的议题和拉取请求。
-| 限定符 | 示例 |
-| ------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| involves:USERNAME
| **[involves:defunkt involves:jlord](https://github.com/search?q=involves%3Adefunkt+involves%3Ajlord&type=Issues)** 匹配涉及 @defunkt 或 @jlord 的议题。 |
-| | [**NOT bootstrap in:body involves:mdo**](https://github.com/search?q=NOT+bootstrap+in%3Abody+involves%3Amdo&type=Issues) 匹配涉及 @mdo 且正文中未包含 "bootstrap" 字样的议题。 |
+| 限定符 | 示例 |
+| ------------------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| involves:USERNAME
| **[involves:defunkt involves:jlord](https://github.com/search?q=involves%3Adefunkt+involves%3Ajlord&type=Issues)** matches issues either @defunkt or @jlord are involved in. |
+| | [**NOT bootstrap in:body involves:mdo**](https://github.com/search?q=NOT+bootstrap+in%3Abody+involves%3Amdo&type=Issues) matches issues @mdo is involved in that do not contain the word "bootstrap" in the body. |
{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.20" or currentVersion == "github-ae@latest" %}
### 搜索链接的议题和拉取请求
diff --git a/translations/zh-CN/content/github/setting-up-and-managing-organizations-and-teams/about-your-organization-dashboard.md b/translations/zh-CN/content/github/setting-up-and-managing-organizations-and-teams/about-your-organization-dashboard.md
index 336c5de176eb..557ea854062c 100644
--- a/translations/zh-CN/content/github/setting-up-and-managing-organizations-and-teams/about-your-organization-dashboard.md
+++ b/translations/zh-CN/content/github/setting-up-and-managing-organizations-and-teams/about-your-organization-dashboard.md
@@ -37,8 +37,8 @@ versions:
- 提交拉取请求审查评论。
- 对仓库复刻。
- 创建 wiki 页面。
- - 推送提交。
- - 创建公共仓库。
+ - Pushes commits.{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+ - Creates a public repository.{% endif %}
### 更多信息
diff --git a/translations/zh-CN/content/github/setting-up-and-managing-organizations-and-teams/restricting-repository-visibility-changes-in-your-organization.md b/translations/zh-CN/content/github/setting-up-and-managing-organizations-and-teams/restricting-repository-visibility-changes-in-your-organization.md
index d1dd5bd89711..f383b8846804 100644
--- a/translations/zh-CN/content/github/setting-up-and-managing-organizations-and-teams/restricting-repository-visibility-changes-in-your-organization.md
+++ b/translations/zh-CN/content/github/setting-up-and-managing-organizations-and-teams/restricting-repository-visibility-changes-in-your-organization.md
@@ -9,7 +9,7 @@ versions:
github-ae: '*'
---
-您可以将更改仓库可见性的权限仅限于组织所有者,或者允许具有仓库管理员权限的成员在私有与公开之间更改仓库可见性。
+You can restrict the ability to change repository visibility to organization owners only, or allow members with admin privileges for a repository to also change visibility.
{% warning %}
@@ -24,3 +24,7 @@ versions:
{% data reusables.organizations.member-privileges %}
5. 在“Repository visibility change(仓库可见性更改)”下,取消选择 **Allow members to change repository visibilities for this organization(允许成员为此组织更改仓库可见性)**。 
6. 单击 **Save(保存)**。
+
+### 延伸阅读
+
+- "[关于仓库可见性](/github/creating-cloning-and-archiving-repositories/about-repository-visibility)"
diff --git a/translations/zh-CN/content/github/setting-up-and-managing-organizations-and-teams/reviewing-the-audit-log-for-your-organization.md b/translations/zh-CN/content/github/setting-up-and-managing-organizations-and-teams/reviewing-the-audit-log-for-your-organization.md
index 12d028716278..1c3a69af505d 100644
--- a/translations/zh-CN/content/github/setting-up-and-managing-organizations-and-teams/reviewing-the-audit-log-for-your-organization.md
+++ b/translations/zh-CN/content/github/setting-up-and-managing-organizations-and-teams/reviewing-the-audit-log-for-your-organization.md
@@ -397,10 +397,10 @@ For more information, see "[Restricting publication of {% data variables.product
| Action | Description
|------------------|-------------------
-| `access` | Triggered when a repository owned by an organization is [switched from "private" to "public"](/articles/making-a-private-repository-public) (or vice versa).
+| `access` | Triggered when a user [changes the visibility](/github/administering-a-repository/setting-repository-visibility) of a repository in the organization.
| `add_member` | Triggered when a user accepts an [invitation to have collaboration access to a repository](/articles/inviting-collaborators-to-a-personal-repository).
| `add_topic` | Triggered when a repository admin [adds a topic](/articles/classifying-your-repository-with-topics) to a repository.
-| `archived` | Triggered when a repository admin [archives a repository](/articles/about-archiving-repositories).{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
+| `archived` | Triggered when a repository admin [archives a repository](/articles/about-archiving-repositories).{% if enterpriseServerVersions contains currentVersion %}
| `config.disable_anonymous_git_access` | Triggered when [anonymous Git read access is disabled](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository) in a public repository.
| `config.enable_anonymous_git_access` | Triggered when [anonymous Git read access is enabled](/enterprise/{{ currentVersion }}/user/articles/enabling-anonymous-git-read-access-for-a-repository) in a public repository.
| `config.lock_anonymous_git_access` | Triggered when a repository's [anonymous Git read access setting is locked](/enterprise/{{ currentVersion }}/admin/guides/user-management/preventing-users-from-changing-anonymous-git-read-access).
@@ -428,9 +428,9 @@ For more information, see "[Restricting publication of {% data variables.product
| Action | Description
|------------------|-------------------
| `close` | Triggered when someone closes a security advisory. For more information, see "[About {% data variables.product.prodname_dotcom %} Security Advisories](/github/managing-security-vulnerabilities/about-github-security-advisories)."
-| `cve_request` | Triggered when someone requests a CVE (Common Vulnerabilities and Exposures) number from {% data.variables.product.prodname_dotcom %} for a draft security advisory.
-| `github_broadcast` | Triggered when {% data.variables.product.prodname_dotcom %} makes a security advisory public in the {% data variables.product.prodname_advisory_database %}.
-| `github_withdraw` | Triggered when {% data.variables.product.prodname_dotcom %} withdraws a security advisory that was published in error.
+| `cve_request` | Triggered when someone requests a CVE (Common Vulnerabilities and Exposures) number from {% data variables.product.prodname_dotcom %} for a draft security advisory.
+| `github_broadcast` | Triggered when {% data variables.product.prodname_dotcom %} makes a security advisory public in the {% data variables.product.prodname_advisory_database %}.
+| `github_withdraw` | Triggered when {% data variables.product.prodname_dotcom %} withdraws a security advisory that was published in error.
| `open` | Triggered when someone opens a draft security advisory.
| `publish` | Triggered when someone publishes a security advisory.
| `reopen` | Triggered when someone reopens as draft security advisory.
@@ -474,7 +474,7 @@ For more information, see "[Restricting publication of {% data variables.product
| Action | Description
|------------------|-------------------
-| `authorized_users_teams` | Triggered when an organization owner or a person with admin permissions to the repository updates the list of people or teams authorized to receive {% data variables.product.prodname_dependabot_alerts %} for vulnerable dependencies in the repository. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-dependabot-alerts)."
+| `authorized_users_teams` | Triggered when an organization owner or a person with admin permissions to the repository updates the list of people or teams authorized to receive {% data variables.product.prodname_dependabot_alerts %} for vulnerable dependencies in the repository. For more information, see "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository#granting-access-to-security-alerts)."
| `disable` | Triggered when a repository owner or person with admin access to the repository disables {% data variables.product.prodname_dependabot_alerts %}.
| `enable` | Triggered when a repository owner or person with admin access to the repository enables {% data variables.product.prodname_dependabot_alerts %}.
diff --git a/translations/zh-CN/content/github/setting-up-and-managing-your-enterprise/about-enterprise-accounts.md b/translations/zh-CN/content/github/setting-up-and-managing-your-enterprise/about-enterprise-accounts.md
index 80c0d88e57d2..25efdd44be2d 100644
--- a/translations/zh-CN/content/github/setting-up-and-managing-your-enterprise/about-enterprise-accounts.md
+++ b/translations/zh-CN/content/github/setting-up-and-managing-your-enterprise/about-enterprise-accounts.md
@@ -20,7 +20,7 @@ versions:
- 安全性(单点登录、双重身份验证)
- 与 {% data variables.contact.enterprise_support %} 共享请求和支持包
-{% data reusables.enterprise-accounts.enterprise-accounts-billing %}
+{% data reusables.enterprise-accounts.enterprise-accounts-billing %} For more information about managing your {% data variables.product.prodname_ghe_cloud %} subscription, see "[Viewing the subscription and usage for your enterprise account](/articles/viewing-the-subscription-and-usage-for-your-enterprise-account)." For more information about managing your {% data variables.product.prodname_ghe_server %} billing settings, see "[Managing billing for your enterprise](/admin/overview/managing-billing-for-your-enterprise)."
有关 {% data variables.product.prodname_ghe_cloud %} 与 {% data variables.product.prodname_ghe_server %} 之间差异的更多信息,请参阅“[{% data variables.product.prodname_dotcom %} 的产品](/articles/githubs-products)”。 要升级至 {% data variables.product.prodname_enterprise %} 或开始使用企业帐户,请联系 {% data variables.contact.contact_enterprise_sales %}。
diff --git a/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/about-your-profile.md b/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/about-your-profile.md
index 20664b568f67..45132aa5fe98 100644
--- a/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/about-your-profile.md
+++ b/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/about-your-profile.md
@@ -13,7 +13,7 @@ versions:
您可以在传记中加入您的个人信息,比如您以前工作的地方、您参与过的项目,或者其他人可能想知道的个人兴趣。 更多信息请参阅“[添加传记到个人资料](/articles/personalizing-your-profile/#adding-a-bio-to-your-profile)”。
-{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
{% data reusables.profile.profile-readme %}
@@ -23,19 +23,17 @@ versions:
人们在访问您的个人资料时会看到您的贡献活动时间表,如您打开的议题和拉取请求、您进行的提交,以及您审查的拉取请求。 您可以选择只显示公共贡献或同时包含私人的匿名化贡献。 更多信息请参阅“[在个人资料页面中查看贡献](/articles/viewing-contributions-on-your-profile-page)”或“[在您的个人资料中公开或隐藏私人贡献](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)”。
-他们还可以查看:
+People who visit your profile can also see the following information.
-- 你拥有或参与的仓库和 gists。 您可以通过将仓库和 Gist 固定到个人资料中来展示您的最佳作品。 更多信息请参阅“[将项目嵌入到个人资料](/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile)”。
-- 您标星的仓库。 更多信息请参阅“[使用星标保存仓库](/articles/saving-repositories-with-stars/)”
-- 您在经常参与的组织、仓库和团队中的活动概述。 For more information, see "[Showing an overview of your activity on your profile](/articles/showing-an-overview-of-your-activity-on-your-profile).{% if currentVersion == "free-pro-team@latest" %}
-- 为您参与 {% data variables.product.prodname_arctic_vault %}、{% data variables.product.prodname_sponsors %} 或 {% data variables.product.company_short %} 开发者计划等计划做宣传的徽章。
-- 您是否在使用 {% data variables.product.prodname_pro %}。 更多信息请参阅“[个性化您的个人资料](/articles/personalizing-your-profile)”。{% endif %}
+- 你拥有或参与的仓库和 gists。 {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}You can showcase your best work by pinning repositories and gists to your profile. 更多信息请参阅“[将项目嵌入到个人资料](/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile)”。{% endif %}
+- 您标星的仓库。 For more information, see "[Saving repositories with stars](/articles/saving-repositories-with-stars/)."
+- 您在经常参与的组织、仓库和团队中的活动概述。 For more information, see "[Showing an overview of your activity on your profile](/articles/showing-an-overview-of-your-activity-on-your-profile)."{% if currentVersion == "free-pro-team@latest" %}
+- Badges that show if you use {% data variables.product.prodname_pro %} or participate in programs like the {% data variables.product.prodname_arctic_vault %}, {% data variables.product.prodname_sponsors %}, or the {% data variables.product.company_short %} Developer Program. 更多信息请参阅“[个性化您的个人资料](/github/setting-up-and-managing-your-github-profile/personalizing-your-profile#displaying-badges-on-your-profile)”。{% endif %}
您还可以在个人资料上设置状态,以提供有关您的可用性的信息。 更多信息请参阅“[设置状态](/articles/personalizing-your-profile/#setting-a-status)”。
### 延伸阅读
- "[如何设置我的头像?](/articles/how-do-i-set-up-my-profile-picture)“
-- "[将仓库嵌入到个人资料](/articles/pinning-repositories-to-your-profile)"
- "[在个人资料中公开或隐藏私有贡献](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)"
- "[在个人资料中查看贡献](/articles/viewing-contributions-on-your-profile)"
diff --git a/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme.md b/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme.md
index 39b12b933cba..0eef53fee2df 100644
--- a/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme.md
+++ b/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme.md
@@ -4,7 +4,6 @@ intro: '可以向您的 {% data variables.product.prodname_dotcom %} 个人资
versions:
free-pro-team: '*'
enterprise-server: '>=2.22'
- github-ae: '*'
---
### 关于您的个人资料自述文件
diff --git a/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/personalizing-your-profile.md b/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/personalizing-your-profile.md
index 17bbbd35a085..b15c14b1c24e 100644
--- a/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/personalizing-your-profile.md
+++ b/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/personalizing-your-profile.md
@@ -50,9 +50,9 @@ versions:
在个人资料中添加个人简历,与其他 {% data variables.product.product_name %} 用户共享您自己的信息。 借助 [@提及](/articles/basic-writing-and-formatting-syntax)和表情符号,可以包含您当前或以前的工作经历、工作类型甚至您喜欢的咖啡种类。
-{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" or currentVersion == "github-ae@latest" %}
+{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}
-要以更长和更突出的方式显示有关自己的自定义信息,您还可以使用个人资料自述文件。 有关个人资料自述文件的更多信息,请参阅“[管理个人资料自述文件](/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme)”。
+要以更长和更突出的方式显示有关自己的自定义信息,您还可以使用个人资料自述文件。 For more information, see "[Managing your profile README](/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme)."
{% endif %}
diff --git a/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile.md b/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile.md
index 1beaf1322841..44ec02ad5171 100644
--- a/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile.md
+++ b/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/pinning-items-to-your-profile.md
@@ -7,7 +7,6 @@ redirect_from:
versions:
free-pro-team: '*'
enterprise-server: '*'
- github-ae: '*'
---
您可以嵌入您拥有的或者对其做出了贡献的公共仓库。 对复刻的提交不计为贡献,因此不能嵌入非自己所有的复刻。 更多信息请参阅“[为什么我的贡献没有在我的个人资料中显示?](/articles/why-are-my-contributions-not-showing-up-on-my-profile)”
diff --git a/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/publicizing-or-hiding-your-private-contributions-on-your-profile.md b/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/publicizing-or-hiding-your-private-contributions-on-your-profile.md
index 9ebf05d52f70..729b8dde2612 100644
--- a/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/publicizing-or-hiding-your-private-contributions-on-your-profile.md
+++ b/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/publicizing-or-hiding-your-private-contributions-on-your-profile.md
@@ -1,6 +1,6 @@
---
title: 在个人资料中公开或隐藏您的私人贡献
-intro: '您的 {% data variables.product.product_name %} 个人资料显示过去一年中您的仓库贡献图。 除了显示公共仓库中的活动之外,您还可以选择选择匿名显示私有仓库中的活动。'
+intro: '您的 {% data variables.product.product_name %} 个人资料显示过去一年中您的仓库贡献图。 You can choose to show anonymized activity from {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.19" %}private and internal{% else %}private{% endif %} repositories{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %} in addition to the activity from public repositories{% endif %}.'
redirect_from:
- /articles/publicizing-or-hiding-your-private-contributions-on-your-profile
versions:
diff --git a/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/viewing-contributions-on-your-profile.md b/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/viewing-contributions-on-your-profile.md
index eb67d61ec899..2a8099631b08 100644
--- a/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/viewing-contributions-on-your-profile.md
+++ b/translations/zh-CN/content/github/setting-up-and-managing-your-github-profile/viewing-contributions-on-your-profile.md
@@ -1,6 +1,6 @@
---
title: 在个人资料中查看贡献
-intro: '您的 {% data variables.product.product_name %} 个人资料显示固定仓库以及过去一年中您的仓库贡献图。'
+intro: 'Your {% data variables.product.product_name %} profile shows off {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}your pinned repositories as well as{% endif %} a graph of your repository contributions over the past year.'
redirect_from:
- /articles/viewing-contributions/
- /articles/viewing-contributions-on-your-profile-page/
@@ -11,7 +11,7 @@ versions:
github-ae: '*'
---
-您的贡献图显示公共仓库的活动。 您可以选择显示公共和私有仓库的活动,并将私有仓库中活动的具体详细信息匿名化。 更多信息请参阅“[在个人资料中公开或隐藏私有贡献](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)”。
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}Your contribution graph shows activity from public repositories. {% endif %}You can choose to show activity from {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}both public and{% endif %}private repositories, with specific details of your activity in private repositories anonymized. 更多信息请参阅“[在个人资料中公开或隐藏私有贡献](/articles/publicizing-or-hiding-your-private-contributions-on-your-profile)”。
{% note %}
@@ -33,16 +33,20 @@ versions:
### 受欢迎的仓库
-此部分显示具有最多查看者的仓库。 您[将仓库固定到个人资料](/articles/pinning-repositories-to-your-profile)后,此部分将更改为“Pinned repositories(固定的仓库)”。
+此部分显示具有最多查看者的仓库。 {% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}Once you [pin repositories to your profile](/articles/pinning-repositories-to-your-profile), this section will change to "Pinned repositories."{% endif %}

+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
### 固定的仓库
此部分显示最多六个公共仓库,并可包括您的仓库以及您对其做出贡献的仓库。 为便于查看关于您选择提供的仓库的重要详细信息,此部分中的每个仓库均包括所进行工作的摘要、仓库已收到的[星号](/articles/saving-repositories-with-stars/)数量以及仓库中使用的主要编程语言。 更多信息请参阅“[将仓库固定到个人资料](/articles/pinning-repositories-to-your-profile)”。

+{% endif %}
+
### 贡献日历
您的贡献日历会显示贡献活动。
diff --git a/translations/zh-CN/content/github/setting-up-and-managing-your-github-user-account/about-your-personal-dashboard.md b/translations/zh-CN/content/github/setting-up-and-managing-your-github-user-account/about-your-personal-dashboard.md
index 9abb9490248d..882d6ed7f6fe 100644
--- a/translations/zh-CN/content/github/setting-up-and-managing-your-github-user-account/about-your-personal-dashboard.md
+++ b/translations/zh-CN/content/github/setting-up-and-managing-your-github-user-account/about-your-personal-dashboard.md
@@ -15,7 +15,7 @@ versions:
个人仪表板是登录 {% data variables.product.product_name %} 时显示的第一页。
-登录后要访问个人仪表板,请单击 {% data variables.product.product_url %} 上任何页面左上角的 {% octicon "mark-github" aria-label="The github octocat logo" %}。
+登录后要访问个人仪表板,请单击 {% data variables.product.product_name %} 上任何页面左上角的 {% octicon "mark-github" aria-label="The github octocat logo" %}。
### 查找近期活动
@@ -39,11 +39,11 @@ The list of top repositories is automatically generated, and can include any rep
当您关注的用户执行以下操作时,您会在消息馈送中看到更新:
- 对仓库标星。
-- 关注另一用户。
-- 创建公共仓库。
+- Follows another user.{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- Creates a public repository.{% endif %}
- 在您关注的仓库上打开具有“需要帮助”或“良好的第一个议题”标签的议题或拉取请求。
-- 推送提交到您关注的仓库。
-- 对公共仓库复刻。
+- Pushes commits to a repository you watch.{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+- Forks a public repository.{% endif %}
有关对仓库标星和关注人员的更多信息,请参阅“[使用星标保存仓库](/articles/saving-repositories-with-stars/)" and "[关注人员](/articles/following-people)”。
diff --git a/translations/zh-CN/content/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository.md b/translations/zh-CN/content/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository.md
index 877b25e81673..6be9f1153d98 100644
--- a/translations/zh-CN/content/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository.md
+++ b/translations/zh-CN/content/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository.md
@@ -50,7 +50,7 @@ versions:
### 延伸阅读
-- "[用户帐户仓库的权限级别](/articles/permission-levels-for-a-user-account-repository/#collaborator-access-on-a-repository-owned-by-a-user-account)"
+- “[用户帐户仓库的权限级别](/articles/permission-levels-for-a-user-account-repository/#collaborator-access-for-a-repository-owned-by-a-user-account)”
- "[从个人仓库删除协作者](/articles/removing-a-collaborator-from-a-personal-repository)"
- "[从协作者的仓库删除您自己](/articles/removing-yourself-from-a-collaborator-s-repository)"
- "[将成员组织成团队](/articles/organizing-members-into-teams)"
diff --git a/translations/zh-CN/content/github/setting-up-and-managing-your-github-user-account/permission-levels-for-a-user-account-repository.md b/translations/zh-CN/content/github/setting-up-and-managing-your-github-user-account/permission-levels-for-a-user-account-repository.md
index 3cfc6af82661..ce484af5e468 100644
--- a/translations/zh-CN/content/github/setting-up-and-managing-your-github-user-account/permission-levels-for-a-user-account-repository.md
+++ b/translations/zh-CN/content/github/setting-up-and-managing-your-github-user-account/permission-levels-for-a-user-account-repository.md
@@ -1,6 +1,6 @@
---
title: 用户帐户仓库的权限级别
-intro: '用户帐户拥有的仓库有两种权限级别:*仓库所有者*和*协作者*。'
+intro: 'A repository owned by a user account has two permission levels: the repository owner and collaborators.'
redirect_from:
- /articles/permission-levels-for-a-user-account-repository
versions:
@@ -9,38 +9,48 @@ versions:
github-ae: '*'
---
+### About permissions levels for a user account repository
+
+Repositories owned by user accounts have one owner. Ownership permissions can't be shared with another user account.
+
+You can also {% if currentVersion == "free-pro-team@latest" %}invite{% else %}add{% endif %} users on {% data variables.product.product_name %} to your repository as collaborators. For more information, see "[Inviting collaborators to a personal repository](/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository)."
+
{% tip %}
-**提示:**如果需要对用户帐户拥有的仓库实施更细致的读/写权限,请考虑将仓库转让给组织。 更多信息请参阅“[转让仓库](/articles/transferring-a-repository)”。
+**Tip:** If you require more granular access to a repository owned by your user account, consider transferring the repository to an organization. 更多信息请参阅“[转让仓库](/github/administering-a-repository/transferring-a-repository#transferring-a-repository-owned-by-your-user-account)”。
{% endtip %}
-#### 所有者对用户帐户拥有的仓库的访问权限
-
-仓库所有者对仓库具有完全控制权。 除了仓库协作者的所有权限之外,仓库所有者还可以:
-
-- {% if currentVersion == "free-pro-team@latest" %}[Invite collaborators](/articles/inviting-collaborators-to-a-personal-repository){% else %}[Add collaborators](/articles/inviting-collaborators-to-a-personal-repository){% endif %}
-- Change the visibility of the repository (from [public to private](/articles/making-a-public-repository-private), or from [private to public](/articles/making-a-private-repository-public)){% if currentVersion == "free-pro-team@latest" %}
-- [限制与仓库的交互](/articles/limiting-interactions-with-your-repository){% endif %}
-- 合并受保护分支上的拉取请求(即使没有批准审查)
-- [删除仓库](/articles/deleting-a-repository)
-- [Manage a repository's topics](/articles/classifying-your-repository-with-topics){% if currentVersion == "free-pro-team@latest" %}
-- 管理安全和分析设置。 For more information, see "[Managing security and analysis settings for your user account](/github/setting-up-and-managing-your-github-user-account/managing-security-and-analysis-settings-for-your-user-account)."{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-- [Enable the dependency graph](/github/visualizing-repository-data-with-graphs/exploring-the-dependencies-and-dependents-of-a-repository) for a private repository{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-- 删除包。 更多信息请参阅“[删除包](/github/managing-packages-with-github-packages/deleting-a-package)”。{% endif %}
-- 创建和编辑仓库社交卡。 更多信息请参阅“[自定义仓库的社交媒体审查](/articles/customizing-your-repositorys-social-media-preview)”。
-- 将仓库设为模板。 For more information, see "[Creating a template repository](/articles/creating-a-template-repository)."{% if currentVersion != "github-ae@latest" %}
-- Receive [{% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot_alerts %}{% else %}security alerts{% endif %} for vulnerable dependencies](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies) in a repository.{% endif %}{% if currentVersion == "free-pro-team@latest" %}
-- 忽略仓库中的 {% data variables.product.prodname_dependabot_alerts %}。 更多信息请参阅“[查看和更新仓库中的漏洞依赖项](/github/managing-security-vulnerabilities/viewing-and-updating-vulnerable-dependencies-in-your-repository)”。
-- [管理私有仓库的数据使用](/github/understanding-how-github-uses-and-protects-your-data/managing-data-use-settings-for-your-private-repository){% endif %}
-- [定义仓库的代码所有者](/articles/about-code-owners)
-- [Archive repositories](/articles/about-archiving-repositories){% if currentVersion == "free-pro-team@latest" %}
-- 创建安全通告。 更多信息请参阅“[关于 {% data variables.product.prodname_security_advisories %}](/github/managing-security-vulnerabilities/about-github-security-advisories)”。
-- 显示赞助按钮。 更多信息请参阅“[在仓库中显示赞助按钮](/articles/displaying-a-sponsor-button-in-your-repository)”。{% endif %}
-
-用户帐户拥有的仓库只有**一个所有者**,此权限无法与其他用户帐户共享。 要将仓库的所有权转让给其他用户,请参阅“[如何转让仓库](/articles/how-to-transfer-a-repository)”。
-
-#### 协作者对用户帐户拥有的仓库的访问权限
+### Owner access for a repository owned by a user account
+
+仓库所有者对仓库具有完全控制权。 In addition to the actions that any collaborator can perform, the repository owner can perform the following actions.
+
+| 操作 | 更多信息 |
+|:---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |:----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| {% if currentVersion == "free-pro-team@latest" %}Invite collaborators{% else %}Add collaborators{% endif %} | |
+| "[邀请个人仓库的协作者](/github/setting-up-and-managing-your-github-user-account/inviting-collaborators-to-a-personal-repository)" | |
+| Change the visibility of the repository | "[Setting repository visibility](/github/administering-a-repository/setting-repository-visibility)" |{% if currentVersion == "free-pro-team@latest" %}
+| Limit interactions with the repository | "[Limiting interactions in your repository](/github/building-a-strong-community/limiting-interactions-in-your-repository)" |{% endif %}
+| 合并受保护分支上的拉取请求(即使没有批准审查) | "[关于受保护分支](/github/administering-a-repository/about-protected-branches)" |
+| 删除仓库 | "[删除仓库](/github/administering-a-repository/deleting-a-repository)" |
+| Manage the repository's topics | "[Classifying your repository with topics](/github/administering-a-repository/classifying-your-repository-with-topics)" |{% if currentVersion == "free-pro-team@latest" %}
+| Manage security and analysis settings for the repository | "[Managing security and analysis settings for your repository](/github/administering-a-repository/managing-security-and-analysis-settings-for-your-repository)" |{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| Enable the dependency graph for a private repository | "[Exploring the dependencies of a repository](/github/visualizing-repository-data-with-graphs/exploring-the-dependencies-of-a-repository#enabling-and-disabling-the-dependency-graph-for-a-private-repository)" |{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| 删除包 | "[Deleting a package](/github/managing-packages-with-github-packages/deleting-a-package)" |{% endif %}
+| Customize the repository's social media preview | "[Customizing your repository's social media preview](/github/administering-a-repository/customizing-your-repositorys-social-media-preview)" |
+| Create a template from the repository | "[Creating a template repository](/github/creating-cloning-and-archiving-repositories/creating-a-template-repository)" |{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+| Receive | |
+| {% if currentVersion == "free-pro-team@latest" or currentVersion ver_gt "enterprise-server@2.21" %}{% data variables.product.prodname_dependabot_alerts %}{% else %}security alerts{% endif %} for vulnerable dependencies | "[About alerts for vulnerable dependencies](/github/managing-security-vulnerabilities/about-alerts-for-vulnerable-dependencies)" |{% endif %}{% if currentVersion == "free-pro-team@latest" %}
+| Dismiss {% data variables.product.prodname_dependabot_alerts %} in the repository | "[查看和更新仓库中的漏洞依赖项](/github/managing-security-vulnerabilities/viewing-and-updating-vulnerable-dependencies-in-your-repository)" |
+| Manage data use for a private repository | "[Managing data use settings for your private repository](/github/understanding-how-github-uses-and-protects-your-data/managing-data-use-settings-for-your-private-repository)"|{% endif %}
+| 定义仓库的代码所有者 | "[关于代码所有者](/github/creating-cloning-and-archiving-repositories/about-code-owners)" |
+| Archive the repository | "[About archiving repositories](/github/creating-cloning-and-archiving-repositories/about-archiving-repositories)" |{% if currentVersion == "free-pro-team@latest" %}
+| Create security advisories | "[关于 {% data variables.product.prodname_security_advisories %}](/github/managing-security-vulnerabilities/about-github-security-advisories)" |
+| Display a sponsor button | "[Displaying a sponsor button in your repository](/github/administering-a-repository/displaying-a-sponsor-button-in-your-repository)" |{% endif %}
+
+### Collaborator access for a repository owned by a user account
+
+Collaborators on a personal repository can pull (read) the contents of the repository and push (write) changes to the repository.
{% note %}
@@ -48,27 +58,26 @@ versions:
{% endnote %}
-个人仓库的协作者可以:
-
-- 推送(写入)、拉取(读取)和复刻(复制)仓库
-- 创建、应用和删除标签及里程碑
-- 打开、关闭、重新打开和分配议题
-- 编辑和删除对提交、拉取请求和议题的评论
-- 将议题或拉取请求标记为重复。 更多信息请参阅“[关于重复的议题和拉取请求](/articles/about-duplicate-issues-and-pull-requests)”。
-- 打开、合并和关闭拉取请求
-- 对拉取请求应用提议的更改。 更多信息请参阅“[合并拉取请求中的反馈](/articles/incorporating-feedback-in-your-pull-request)”。
-- Send pull requests from forks of the repository{% if currentVersion == "free-pro-team@latest" %}
-- 发布、查看和安装包。 更多信息请参阅“[发布和管理包](/github/managing-packages-with-github-packages/publishing-and-managing-packages)”。{% endif %}
-- 创建和编辑 Wiki
-- 创建和编辑发行版. 更多信息请参阅“[管理仓库中的发行版](/github/administering-a-repository/managing-releases-in-a-repository)”。
-- 作为仓库协作者删除自己
-- 提交会影响其合并性的拉取请求审查
-- 作为仓库的指定代码所有者。 更多信息请参阅“[关于代码所有者](/articles/about-code-owners)”。
-- 锁定对话。 For more information, see "[Locking conversations](/articles/locking-conversations)."{% if currentVersion == "free-pro-team@latest" %}
-- 向 {% data variables.contact.contact_support %} 报告滥用的内容。 更多信息请参阅“[报告滥用或垃圾邮件](/articles/reporting-abuse-or-spam)”。{% endif %}
-- 将议题转让给不同的仓库 更多信息请参阅“[将议题传输到其他仓库](/articles/transferring-an-issue-to-another-repository)”。
+Collaborators can also perform the following actions.
+
+| 操作 | 更多信息 |
+|:----------------------------------------------------------------------------------------- |:------------------------------------------------------------------------------------------------------------------------------------------------------------ |
+| Fork the repository | "[关于复刻](/github/collaborating-with-issues-and-pull-requests/about-forks)" |
+| Create, edit, and delete comments on commits, pull requests, and issues in the repository | - "[About issues](/github/managing-your-work-on-github/about-issues)"
- "[Commenting on a pull request](/github/collaborating-with-issues-and-pull-requests/commenting-on-a-pull-request)"
- "[Managing disruptive comments](/github/building-a-strong-community/managing-disruptive-comments)"
|
+| Create, assign, close, and re-open issues in the repository | "[Managing your work with issues](/github/managing-your-work-on-github/managing-your-work-with-issues)" |
+| Manage labels for issues and pull requests in the repository | "[Labeling issues and pull requests](/github/managing-your-work-on-github/labeling-issues-and-pull-requests)" |
+| Manage milestones for issues and pull requests in the repository | "[创建和编辑议题及拉取请求的里程碑](/github/managing-your-work-on-github/creating-and-editing-milestones-for-issues-and-pull-requests)" |
+| Mark an issue or pull request in the repository as a duplicate | "[About duplicate issues and pull requests](/github/managing-your-work-on-github/about-duplicate-issues-and-pull-requests)" |
+| Create, merge, and close pull requests in the repository | "[Proposing changes to your work with pull requests](/github/collaborating-with-issues-and-pull-requests/proposing-changes-to-your-work-with-pull-requests)" |
+| Apply suggested changes to pull requests in the repository | "[Incorporating feedback in your pull request](/github/collaborating-with-issues-and-pull-requests/incorporating-feedback-in-your-pull-request)" |
+| Create a pull request from a fork of the repository | "[从复刻创建拉取请求](/github/collaborating-with-issues-and-pull-requests/creating-a-pull-request-from-a-fork)" |
+| Submit a review on a pull request that affects the mergeability of the pull request | "[审查拉取请求中提议的更改](/github/collaborating-with-issues-and-pull-requests/reviewing-proposed-changes-in-a-pull-request)" |
+| Create and edit a wiki for the repository | "[关于 wikis](/github/building-a-strong-community/about-wikis)" |
+| Create and edit releases for the repository | "[Managing releases in a repository](/github/administering-a-repository/managing-releases-in-a-repository)" |
+| Act as a code owner for the repository | "[About code owners](/articles/about-code-owners)" |{% if currentVersion == "free-pro-team@latest" %}
+| Publish, view, or install packages | "[Publishing and managing packages](/github/managing-packages-with-github-packages/publishing-and-managing-packages)" |{% endif %}
+| 作为仓库协作者删除自己 | "[从协作者的仓库删除您自己](/github/setting-up-and-managing-your-github-user-account/removing-yourself-from-a-collaborators-repository)" |
### 延伸阅读
-- "[邀请个人仓库的协作者](/articles/inviting-collaborators-to-a-personal-repository)"
- "[组织的仓库权限级别](/articles/repository-permission-levels-for-an-organization)"
diff --git a/translations/zh-CN/content/github/site-policy/github-marketplace-terms-of-service.md b/translations/zh-CN/content/github/site-policy/github-marketplace-terms-of-service.md
index 93847efbee7f..5229beb21be8 100644
--- a/translations/zh-CN/content/github/site-policy/github-marketplace-terms-of-service.md
+++ b/translations/zh-CN/content/github/site-policy/github-marketplace-terms-of-service.md
@@ -6,11 +6,11 @@ versions:
free-pro-team: '*'
---
-欢迎使用 GitHub Marketplace ("Marketplace")! 我们很高兴在这里与您邂逅。 在访问或使用 GitHub Marketplace 之前,请仔细阅读这些服务条款(“Marketplace 条款”)。 GitHub Marketplace 是一个供您购买(免费或付费)可用于 GitHub.com 帐户的开发者产品(“开发者产品”)的平台。 开发者产品虽然通过 GitHub, Inc. ("GitHub"、“我们”)出售,但可能由 GitHub 或第三方软件提供者开发和维护,并可能需要您同意单独的服务条款 。 使用和/或购买开发者产品需遵守这些 Marketplace 条款,需支付适用的费用,可能还需要遵守该开发者产品的第三方许可者(“产品提供者”)规定的其他条款。
+欢迎使用 GitHub Marketplace ("Marketplace")! 我们很高兴在这里与您邂逅。 在访问或使用 GitHub Marketplace 之前,请仔细阅读这些服务条款(“Marketplace 条款”)。 GitHub Marketplace is a platform that allows you to select developer apps or actions (for free or for a charge) that can be used with your GitHub.com account ("Developer Products"). Although offered by GitHub, Inc. ("GitHub", "we", "us"), Developer Products may be developed and maintained by either GitHub or by third-party software providers. Your selection or use of Developer Products is subject to these Marketplace Terms and any applicable fees, and may require you to agree to additional terms as provided by the third party licensor of that Developer Product (the "Product Provider").
使用 Marketplace,即表示您同意遵守这些 Marketplace 条款。
-生效日期:2017 年 10 月 11 日
+Effective Date: November 20, 2020
### A. GitHub.com 的服务条款
@@ -50,11 +50,11 @@ Marketplace 由 GitHub 提供,受
### E. 您的数据和 GitHub 隐私政策
-**隐私。**当您购买或订阅开发者产品时,GitHub 必须与产品提供者分享某些个人信息(定义见 GitHub 隐私声明),以便为您提供开发者产品,无论您的隐私设置如何。 GitHub 分享信息的多少取决于您所选开发者产品的要求,最少会分享您的用户帐户名称、ID 和主电子邮件地址,最多会分享您仓库内容的访问权限,包括阅读和修改您私有数据的权限。 通过 OAuth 授予权限时,您可以查看开发者产品要求的权限范围,然后选择接受或拒绝。 根据 [GitHub 隐私声明](/articles/github-privacy-statement/),我们只向产品提供者提供完成交易必需的最少量信息。
+**Privacy.** When you select or use a Developer Product, GitHub may share certain Personal Information (as defined in the [GitHub Privacy Statement](/articles/github-privacy-statement/)) with the Product Provider (if any such Personal Information is received from you) in order to provide you with the Developer Product, regardless of your privacy settings. GitHub 分享信息的多少取决于您所选开发者产品的要求,最少会分享您的用户帐户名称、ID 和主电子邮件地址,最多会分享您仓库内容的访问权限,包括阅读和修改您私有数据的权限。 通过 OAuth 授予权限时,您可以查看开发者产品要求的权限范围,然后选择接受或拒绝。
-如果您通过帐户设置取消开发者产品服务并撤销访问权限,则产品提供者将无法再访问您的帐户。 产品提供者负责在其规定的时间内从其系统中删除您的个人信息。 请联系产品提供者以确保您的帐户以被正确终止。
+If you cease using the Developer Product and revoke access through your account settings, the Product Provider will no longer be able to access your account. 产品提供者负责在其规定的时间内从其系统中删除您的个人信息。 请联系产品提供者以确保您的帐户以被正确终止。
-**数据安全免责声明。** 当您购买或订阅开发者产品时, 开发者产品的安全性及其对您数据的保管是产品提供者的责任。 您有责任出于自己的安全性 、风险和合规性考虑,了解购买和使用开发者产品的相关安全因素。
+**Data Security and Privacy Disclaimer.** When you select or use a Developer Product, the security of the Developer Product and the custodianship of your data, including your Personal Information (if any), is the responsibility of the Product Provider. You are responsible for understanding the security and privacy considerations of the selection or use of the Developer Product for your own security and privacy risk and compliance considerations.
diff --git a/translations/zh-CN/content/github/using-git/setting-your-username-in-git.md b/translations/zh-CN/content/github/using-git/setting-your-username-in-git.md
index 95cb456dcefc..1bdda93e02c0 100644
--- a/translations/zh-CN/content/github/using-git/setting-your-username-in-git.md
+++ b/translations/zh-CN/content/github/using-git/setting-your-username-in-git.md
@@ -20,13 +20,13 @@ versions:
2. {% data reusables.user_settings.set_your_git_username %}
```shell
$ git config --global user.name "Mona Lisa"
- ```
+ ```
3. {% data reusables.user_settings.confirm_git_username_correct %}
```shell
$ git config --global user.name
> Mona Lisa
- ```
+ ```
### 为一个仓库设置 Git 用户名
@@ -37,13 +37,13 @@ versions:
3. {% data reusables.user_settings.set_your_git_username %}
```shell
$ git config user.name "Mona Lisa"
- ```
+ ```
3. {% data reusables.user_settings.confirm_git_username_correct %}
```shell
$ git config user.name
> Mona Lisa
- ```
+ ```
### 延伸阅读
diff --git a/translations/zh-CN/content/github/using-git/which-remote-url-should-i-use.md b/translations/zh-CN/content/github/using-git/which-remote-url-should-i-use.md
index b4e1bc63dea4..c198e7771045 100644
--- a/translations/zh-CN/content/github/using-git/which-remote-url-should-i-use.md
+++ b/translations/zh-CN/content/github/using-git/which-remote-url-should-i-use.md
@@ -3,7 +3,7 @@ title: 我应使用哪个远程 URL?
redirect_from:
- /articles/which-url-should-i-use/
- /articles/which-remote-url-should-i-use
-intro: '克隆 {% data variables.product.prodname_dotcom %} 上的仓库有几种方法。'
+intro: '克隆 {% data variables.product.product_location %} 上的仓库有几种方法。'
versions:
free-pro-team: '*'
enterprise-server: '*'
@@ -16,7 +16,7 @@ versions:
### 使用 HTTPS URL 克隆
-`https://` 克隆 URL 在所有仓库(公共和私有)中提供。 即使您在防火墙或代理后面,这些 URL 也有效。
+The `https://` clone URLs are available on all repositories, regardless of visibility. `https://` clone URLs work even if you are behind a firewall or proxy.
当您在命令行中使用 HTTPS URL 对远程仓库执行 `git clone`、`git fetch`、`git pull` 或 `git push` 命令时,Git 将要求您输入 {% data variables.product.product_name %} 用户名和密码。 {% data reusables.user_settings.password-authentication-deprecation %}
diff --git a/translations/zh-CN/content/github/writing-on-github/creating-gists.md b/translations/zh-CN/content/github/writing-on-github/creating-gists.md
index 2f93de0fee81..be81e726ab29 100644
--- a/translations/zh-CN/content/github/writing-on-github/creating-gists.md
+++ b/translations/zh-CN/content/github/writing-on-github/creating-gists.md
@@ -33,8 +33,12 @@ Secret gists don't show up in {% data variables.gists.discover_url %} and are no
- 有人在 gist 中提及您。
- 您单击任何 gist 顶部的 **Subscribe(订阅)**订阅了 gist。
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
您可以在个人资料中置顶 Gist,使其他人更容易看到它们。 更多信息请参阅“[将项目嵌入到个人资料](/articles/pinning-items-to-your-profile)”。
+{% endif %}
+
您可以到 {% data variables.gists.gist_homepage %} 单击 **All Gists(所有 Gists)**发现其他人创建的 gists。 将会显示所有 gists 存储的页面,gist 按创建或更新时间显示。 您也可以通过 {% data variables.gists.gist_search_url %} 按语言搜索 gist。 Gist 搜索使用的搜索语法与[代码搜索](/articles/searching-code)相同。
由于 gists 是 Git 仓库,因此您可以查看其整个提交历史记录,包括差异。 您也可以复刻或克隆 gists。 更多信息请参阅[“复刻和克隆 gists”](/articles/forking-and-cloning-gists)。
diff --git a/translations/zh-CN/content/packages/managing-container-images-with-github-container-registry/deleting-a-container-image.md b/translations/zh-CN/content/packages/managing-container-images-with-github-container-registry/deleting-a-container-image.md
index ebf4ca347e4a..cfc088b92eca 100644
--- a/translations/zh-CN/content/packages/managing-container-images-with-github-container-registry/deleting-a-container-image.md
+++ b/translations/zh-CN/content/packages/managing-container-images-with-github-container-registry/deleting-a-container-image.md
@@ -16,10 +16,6 @@ versions:
删除公共包时,请注意,您可能会破坏依赖于包的项目。
-### 保留的包版本和名称
-
-{% data reusables.package_registry.package-immutability %}
-
### 删除 {% data variables.product.prodname_dotcom %} 上用户拥有的容器映像版本
{% data reusables.package_registry.package-settings-from-user-level %}
diff --git a/translations/zh-CN/content/packages/publishing-and-managing-packages/deleting-a-package.md b/translations/zh-CN/content/packages/publishing-and-managing-packages/deleting-a-package.md
index 9ec33a70d9bf..044539cfa7a8 100644
--- a/translations/zh-CN/content/packages/publishing-and-managing-packages/deleting-a-package.md
+++ b/translations/zh-CN/content/packages/publishing-and-managing-packages/deleting-a-package.md
@@ -35,10 +35,6 @@ versions:
{% endif %}
-### 保留的包版本和名称
-
-{% data reusables.package_registry.package-immutability %}
-
### 在 {% data variables.product.product_name %} 上删除私有包的版本
要删除私有包版本,您必须具有仓库的管理员权限。
diff --git a/translations/zh-CN/content/packages/publishing-and-managing-packages/publishing-a-package.md b/translations/zh-CN/content/packages/publishing-and-managing-packages/publishing-a-package.md
index 3eece7e742d7..69b92e712f45 100644
--- a/translations/zh-CN/content/packages/publishing-and-managing-packages/publishing-a-package.md
+++ b/translations/zh-CN/content/packages/publishing-and-managing-packages/publishing-a-package.md
@@ -18,8 +18,6 @@ versions:
{% data reusables.package_registry.public-or-private-packages %} 一个仓库可包含多个包。 为避免混淆,请确保使用自述文件和说明清楚地阐明每个包的相关信息。
-{% data reusables.package_registry.package-immutability %}
-
{% if currentVersion == "free-pro-team@latest" %}
如果软件包的新版本修复了安全漏洞,您应该在仓库中发布安全通告。
{% data variables.product.prodname_dotcom %} 审查每个发布的安全通告,并且可能使用它向受影响的仓库发送 {% data variables.product.prodname_dependabot_alerts %}。 更多信息请参阅“[关于 GitHub 安全通告](/github/managing-security-vulnerabilities/about-github-security-advisories)”。
diff --git a/translations/zh-CN/content/packages/using-github-packages-with-your-projects-ecosystem/configuring-npm-for-use-with-github-packages.md b/translations/zh-CN/content/packages/using-github-packages-with-your-projects-ecosystem/configuring-npm-for-use-with-github-packages.md
index 9f326abbf399..779ef2ef3c41 100644
--- a/translations/zh-CN/content/packages/using-github-packages-with-your-projects-ecosystem/configuring-npm-for-use-with-github-packages.md
+++ b/translations/zh-CN/content/packages/using-github-packages-with-your-projects-ecosystem/configuring-npm-for-use-with-github-packages.md
@@ -48,12 +48,15 @@ $ npm login --registry=https://npm.pkg.github.com
要通过登录到 npm 进行身份验证,请使用 `npm login` 命令,将 *USERNAME* 替换为您的 {% data variables.product.prodname_dotcom %} 用户名,将 *TOKEN* 替换为您的个人访问令牌,将 *PUBLIC-EMAIL-ADDRESS* 替换为您的电子邮件地址。
+If {% data variables.product.prodname_registry %} is not your default package registry for using npm and you want to use the `npm audit` command, we recommend you use the `--scope` flag with the owner of the package when you authenticate to {% data variables.product.prodname_registry %}.
+
{% if enterpriseServerVersions contains currentVersion %}
有关创建包的更多信息,请参阅 [maven.apache.org 文档](https://maven.apache.org/guides/getting-started/maven-in-five-minutes.html)。
{% endif %}
```shell
-$ npm login --registry=https://{% if currentVersion == "free-pro-team@latest" %}npm.pkg.github.com{% else %}npm.HOSTNAME/{% endif %}
+$ npm login --scope=@OWNER --registry=https://{% if currentVersion == "free-pro-team@latest" %}npm.pkg.github.com{% else %}npm.HOSTNAME/{% endif %}
+
> Username: USERNAME
> Password: TOKEN
> Email: PUBLIC-EMAIL-ADDRESS
@@ -63,9 +66,10 @@ $ npm login --registry=https://{% if currentVersion == "free-pro-team@latest" %}
例如,*OctodogApp* 和 *OctocatApp* 项目将发布到同一个仓库:
```shell
-registry=https://npm.pkg.github.com/OWNER
-@OWNER:registry=https://npm.pkg.github.com
-@OWNER:registry=https://npm.pkg.github.com
+$ npm login --scope=@OWNER --registry=https://HOSTNAME/_registry/npm/
+> Username: USERNAME
+> Password: TOKEN
+> Email: PUBLIC-EMAIL-ADDRESS
```
{% endif %}
diff --git a/translations/zh-CN/data/reusables/enterprise-accounts/enterprise-accounts-billing.md b/translations/zh-CN/data/reusables/enterprise-accounts/enterprise-accounts-billing.md
index d833c4257b37..174a51adff0b 100644
--- a/translations/zh-CN/data/reusables/enterprise-accounts/enterprise-accounts-billing.md
+++ b/translations/zh-CN/data/reusables/enterprise-accounts/enterprise-accounts-billing.md
@@ -1 +1 @@
-企业帐户目前可用于通过发票付款的 {% data variables.product.prodname_ghe_cloud %} 和 {% data variables.product.prodname_ghe_server %} 客户。 与企业帐户关联的所有组织和 {% data variables.product.prodname_ghe_server %} 实例的帐单将汇总为单个帐单。 有关管理 {% data variables.product.prodname_ghe_cloud %} 订阅的更多信息,请参阅“[查看企业帐户的订阅和使用情况](/articles/viewing-the-subscription-and-usage-for-your-enterprise-account)”。 For more information about managing your {% data variables.product.prodname_ghe_server %} billing settings, see "[Managing billing for your enterprise](/admin/overview/managing-billing-for-your-enterprise)."
+企业帐户目前可用于通过发票付款的 {% data variables.product.prodname_ghe_cloud %} 和 {% data variables.product.prodname_ghe_server %} 客户。 与企业帐户关联的所有组织和 {% data variables.product.prodname_ghe_server %} 实例的帐单将汇总为单个帐单。
diff --git a/translations/zh-CN/data/reusables/enterprise_installation/hardware-considerations-all-platforms.md b/translations/zh-CN/data/reusables/enterprise_installation/hardware-considerations-all-platforms.md
index 2b0663ccfd4f..0a7dc3ed9e1e 100644
--- a/translations/zh-CN/data/reusables/enterprise_installation/hardware-considerations-all-platforms.md
+++ b/translations/zh-CN/data/reusables/enterprise_installation/hardware-considerations-all-platforms.md
@@ -1,4 +1,5 @@
- [最低要求](#minimum-requirements)
+- [{% data variables.product.prodname_ghe_server %} 2.22 中的测试功能](#beta-features-in-github-enterprise-server-222)
- [存储器](#storage)
- [CPU 和内存](#cpu-and-memory)
@@ -6,23 +7,18 @@
建议根据 {% data variables.product.product_location %} 的用户许可数选择不同的硬件配置。 如果预配的资源超过最低要求,您的实例将表现出更好的性能和扩展。
-{% data reusables.enterprise_installation.hardware-rec-table %} 有关为现有实例调整资源的更多信息,请参阅“[增加存储容量](/enterprise/admin/installation/increasing-storage-capacity)”和“[增加 CPU 或内存资源](/enterprise/admin/installation/increasing-cpu-or-memory-resources)”。
-
-{% if currentVersion == "enterprise-server@2.22" or currentVersion == "github-ae@latest" %}
-
-如果您为实例上的 {% data variables.product.prodname_actions %} 启用测试版,建议您规划额外的容量。
+{% data reusables.enterprise_installation.hardware-rec-table %}{% if currentVersion == "enterprise-server@2.22" or currentVersion == "github-ae@latest" %} If you enable the beta for {% data variables.product.prodname_actions %}, review the following requirements and recommendations.
- 您必须为 {% data variables.product.prodname_actions %} 工作流程配置至少一个运行器。 更多信息请参阅“[关于自托管运行器](/actions/hosting-your-own-runners/about-self-hosted-runners)”。
- 您必须配置外部 Blob 存储。 更多信息请参阅“[启用 {% data variables.product.prodname_actions %} 和配置存储](/enterprise/admin/github-actions/enabling-github-actions-and-configuring-storage)”。
-
-需要为实例预配的额外 CPU 和内存资源取决于用户同时运行的工作流程数量,以及用户活动、自动化和集成的总体水平。
-
-| 每分钟最大作业数 | vCPU | 内存 |
-|:-------- | ----:| -------:|
-| 轻型测试 | 4 | 30.5 GB |
-| 25 | 8 | 61 GB |
-| 35 | 16 | 122 GB |
-| 100 | 32 | 244 GB |
+- You may need to configure additional CPU and memory resources. The additional resources you need to provision for {% data variables.product.prodname_actions %} depend on the number of workflows your users run concurrently, and the overall levels of activity for users, automations, and integrations.
+
+ | 每分钟最大作业数 | Additional vCPUs | Additional memory |
+ |:-------- | ----------------:| -----------------:|
+ | 轻型测试 | 4 | 30.5 GB |
+ | 25 | 8 | 61 GB |
+ | 35 | 16 | 122 GB |
+ | 100 | 32 | 244 GB |
{% endif %}
diff --git a/translations/zh-CN/data/reusables/enterprise_installation/hardware-rec-table.md b/translations/zh-CN/data/reusables/enterprise_installation/hardware-rec-table.md
index f9ee0bf5bb80..e528fa16cafc 100644
--- a/translations/zh-CN/data/reusables/enterprise_installation/hardware-rec-table.md
+++ b/translations/zh-CN/data/reusables/enterprise_installation/hardware-rec-table.md
@@ -1,6 +1,12 @@
{% if currentVersion == "enterprise-server@2.22" %}
-启用测试功能的实例的最低要求在下表中用**粗体**表示。 更多信息请参阅“[{% data variables.product.prodname_ghe_server %} 2.22 中的测试功能](#beta-features-in-github-enterprise-server-222)”。 |{% endif %}
+{% note %}
+
+**Note**: If you enable beta features for {% data variables.product.prodname_ghe_server %} 2.22, your instance requires additional hardware resources. 启用测试功能的实例的最低要求在下表中用**粗体**表示。 For more information about the features in beta, see "[Beta features in {% data variables.product.prodname_ghe_server %} 2.22](#beta-features-in-github-enterprise-server-222)."
+
+{% endnote %}
+|
+{% endif %}
| 用户许可 | vCPU | 内存 | 附加的存储容量 | 根存储容量 |
|:----------------- | -------------------------------------------------------------------------------------------------------------------------------------:| ---------------------------------------------------------------------------------------------------------------------------------------------:| ---------------------------------------------------------------------------------------------------------------------------------------------:| ------:|
| 试用版、演示版或 10 个轻度用户 | 2{% if currentVersion == "enterprise-server@2.22" %}
或 [**4**](#beta-features-in-github-enterprise-server-222){% endif %} | 16 GB{% if currentVersion == "enterprise-server@2.22" %}
或 [**32 GB**](#beta-features-in-github-enterprise-server-222){% endif %} | 100 GB{% if currentVersion == "enterprise-server@2.22" %}
或 [**150 GB**](#beta-features-in-github-enterprise-server-222){% endif %} | 200 GB |
@@ -9,8 +15,14 @@
| 5000-8000 | 12{% if currentVersion == "enterprise-server@2.22" %}
或 [**16**](#beta-features-in-github-enterprise-server-222){% endif %} | 96 GB | 750 GB | 200 GB |
| 8000-10000+ | 16{% if currentVersion == "enterprise-server@2.22" %}
或 [**20**](#beta-features-in-github-enterprise-server-222){% endif %} | 128 GB{% if currentVersion == "enterprise-server@2.22" %}
或 [**160 GB**](#beta-features-in-github-enterprise-server-222){% endif %} | 1000 GB | 200 GB |
+For more information about adjusting resources for an existing instance, see "[Increasing storage capacity](/enterprise/admin/installation/increasing-storage-capacity)" and "[Increasing CPU or memory resources](/enterprise/admin/installation/increasing-cpu-or-memory-resources)."
+
{% if currentVersion == "enterprise-server@2.22" %}
#### {% data variables.product.prodname_ghe_server %} 2.22 中的测试功能
-如果您在 {% data variables.product.prodname_ghe_server %} 2.22 中启用测试功能,则您的实例需要额外的硬件资源。 有关测试功能的更多信息,请参阅 {% data variables.product.prodname_enterprise %} 网站上的 [2.22 系列发行说明](https://enterprise.github.com/releases/series/2.22)。{% endif %}
+You can sign up for beta features available in {% data variables.product.prodname_ghe_server %} 2.22 such as {% data variables.product.prodname_actions %}, {% data variables.product.prodname_registry %}, and {% data variables.product.prodname_code_scanning %}. For more information, see the [release notes for the 2.22 series](https://enterprise.github.com/releases/series/2.22#release-2.22.0) on the {% data variables.product.prodname_enterprise %} website.
+
+If you enable beta features for {% data variables.product.prodname_ghe_server %} 2.22, your instance requires additional hardware resources. For more information, see "[Minimum requirements](#minimum-requirements)".
+
+{% endif %}
diff --git a/translations/zh-CN/data/reusables/enterprise_installation/image-urls-viewable-warning.md b/translations/zh-CN/data/reusables/enterprise_installation/image-urls-viewable-warning.md
index 0791033a713f..2a26ad6d64f7 100644
--- a/translations/zh-CN/data/reusables/enterprise_installation/image-urls-viewable-warning.md
+++ b/translations/zh-CN/data/reusables/enterprise_installation/image-urls-viewable-warning.md
@@ -1,5 +1,5 @@
{% warning %}
-**警告:**如果您在拉取请求或议题评论中添加了图像附件,则任何人都可以查看匿名图像 URL,无需身份验证,即使该拉取请求位于私有仓库中或者启用了私有模式。 要对敏感图像保密,请从需要身份验证的私有网络或服务器提供它们。
+**Warning:** If you add an image attachment to a pull request or issue comment, anyone can view the anonymized image URL without authentication{% if enterpriseServerVersions contains currentVersion %}, even if the pull request is in a private repository, or if private mode is enabled. To prevent unauthorized access to the images, ensure that you restrict network access to the systems that serve the images, including {% data variables.product.product_location %}{% endif %}.{% if currentVersion == "github-ae@latest" %} To prevent unauthorized access to image URLs on {% data variables.product.product_name %}, consider restricting network traffic to your enterprise. For more information, see "[Restricting network traffic to your enterprise](/admin/configuration/restricting-network-traffic-to-your-enterprise)."{% endif %}
{% endwarning %}
diff --git a/translations/zh-CN/data/reusables/gated-features/pages.md b/translations/zh-CN/data/reusables/gated-features/pages.md
index 06a1c92dfa65..31caf392d2d3 100644
--- a/translations/zh-CN/data/reusables/gated-features/pages.md
+++ b/translations/zh-CN/data/reusables/gated-features/pages.md
@@ -1 +1 @@
-{% data variables.product.prodname_pages %} 适用于具有 {% data variables.product.prodname_free_user %} 和组织的 {% data variables.product.prodname_free_team %} 的公共仓库,以及具有 {% data variables.product.prodname_pro %}、{% data variables.product.prodname_team %}、{% data variables.product.prodname_ghe_cloud %}、{% if currentVersion == "github-ae@latest" %} {% data variables.product.prodname_ghe_managed %}、{% endif %} 和 {% data variables.product.prodname_ghe_server %} 的公共和私有仓库。 {% if currentVersion == "free-pro-team@latest" %}{% data reusables.gated-features.more-info %}{% endif %}
+{% if currentVersion == "github-ae@latest" %}{% data variables.product.prodname_pages %} is available in internal and private repositories with {% data variables.product.prodname_ghe_managed %}. {% endif %}{% data variables.product.prodname_pages %} is available in public repositories with {% data variables.product.prodname_free_user %} and {% data variables.product.prodname_free_team %} for organizations, and in public and private repositories with {% data variables.product.prodname_pro %}, {% data variables.product.prodname_team %}, {% data variables.product.prodname_ghe_cloud %}, and {% data variables.product.prodname_ghe_server %}. {% if currentVersion == "free-pro-team@latest" %}{% data reusables.gated-features.more-info %}{% endif %}
diff --git a/translations/zh-CN/data/reusables/gated-features/wikis.md b/translations/zh-CN/data/reusables/gated-features/wikis.md
index f470574be627..d7611dc3638c 100644
--- a/translations/zh-CN/data/reusables/gated-features/wikis.md
+++ b/translations/zh-CN/data/reusables/gated-features/wikis.md
@@ -1 +1 @@
-Wikis 适用于具有 {% data variables.product.prodname_free_user %} 和组织的 {% data variables.product.prodname_free_team %} 的公共仓库,以及具有 {% data variables.product.prodname_pro %}、{% data variables.product.prodname_team %}、{% data variables.product.prodname_ghe_cloud %}、{% if currentVersion == "github-ae@latest" %} {% data variables.product.prodname_ghe_managed %}、{% endif %} 和 {% data variables.product.prodname_ghe_server %} 的公共和私有仓库。 {% if currentVersion == "free-pro-team@latest" %}{% data reusables.gated-features.more-info %}{% endif %}
+{% if currentVersion == "github-ae@latest" %}Wikis are available in internal and private repositories with {% data variables.product.prodname_ghe_managed %}.{% endif %} Wikis are available in public repositories with {% data variables.product.prodname_free_user %} and {% data variables.product.prodname_free_team %} for organizations, and in public and private repositories with {% data variables.product.prodname_pro %}, {% data variables.product.prodname_team %}, {% data variables.product.prodname_ghe_cloud %} and {% data variables.product.prodname_ghe_server %}. {% if currentVersion == "free-pro-team@latest" %}{% data reusables.gated-features.more-info %}{% endif %}
diff --git a/translations/zh-CN/data/reusables/github-actions/self-hosted-runner-configure-runner-group-access.md b/translations/zh-CN/data/reusables/github-actions/self-hosted-runner-configure-runner-group-access.md
index 39aecaf8b4dd..9ac93498fa1e 100644
--- a/translations/zh-CN/data/reusables/github-actions/self-hosted-runner-configure-runner-group-access.md
+++ b/translations/zh-CN/data/reusables/github-actions/self-hosted-runner-configure-runner-group-access.md
@@ -1,2 +1,12 @@
-1. 在设置页面的 **Self-hosted runners(自托管运行器)**部分,单击要配置的运行器组旁边的 {% octicon "kebab-horizontal" aria-label="The horizontal kebab icon" %},然后单击 **Edit name and [organization|repository] access(编辑名称和[组织|仓库]权限)**。 
-1. 从下拉列表中选择一个新策略,或修改运行器组名称。
+1. In the **Self-hosted runners** section of the settings page, click {% octicon "kebab-horizontal" aria-label="The horizontal kebab icon" %} next to the runner group you'd like to configure, then click **Edit name and [organization|repository] access**.
+ 
+1. Modify your policy options, or change the runner group name.
+
+ {% warning %}
+
+ **Warning**
+ {% indented_data_reference site.data.reusables.github-actions.self-hosted-runner-security spaces=3 %}
+ For more information, see "[About self-hosted runners](/actions/hosting-your-own-runners/about-self-hosted-runners#self-hosted-runner-security-with-public-repositories)."
+
+ {% endwarning %}
+
diff --git a/translations/zh-CN/data/reusables/notifications-v2/custom-notifications-beta.md b/translations/zh-CN/data/reusables/notifications-v2/custom-notifications-beta.md
new file mode 100644
index 000000000000..dbcee80cfe1d
--- /dev/null
+++ b/translations/zh-CN/data/reusables/notifications-v2/custom-notifications-beta.md
@@ -0,0 +1,9 @@
+{% if currentVersion == "free-pro-team@latest" %}
+
+{% note %}
+
+**Note:** Custom notifications are currently in beta and subject to change.
+
+{% endnote %}
+
+{% endif %}
diff --git a/translations/zh-CN/data/reusables/notifications/outbound_email_tip.md b/translations/zh-CN/data/reusables/notifications/outbound_email_tip.md
index ec8efc0377b3..843ea8a8e066 100644
--- a/translations/zh-CN/data/reusables/notifications/outbound_email_tip.md
+++ b/translations/zh-CN/data/reusables/notifications/outbound_email_tip.md
@@ -1,7 +1,9 @@
-{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
- {% tip %}
+{% if enterpriseServerVersions contains currentVersion %}
- 如果在 {% data variables.product.product_location %} 上启用了出站电子邮件支持,您将只收到邮件通知。 更多信息请联系站点管理员。
+{% note %}
+
+**Note**: You'll only receive email notifications if outbound email support is enabled on {% data variables.product.product_location %}. 更多信息请联系站点管理员。
+
+{% endnote %}
- {% endtip %}
{% endif %}
diff --git a/translations/zh-CN/data/reusables/notifications/shared_state.md b/translations/zh-CN/data/reusables/notifications/shared_state.md
index 2e5e542dbb21..541fa5ae554a 100644
--- a/translations/zh-CN/data/reusables/notifications/shared_state.md
+++ b/translations/zh-CN/data/reusables/notifications/shared_state.md
@@ -1,5 +1,5 @@
{% tip %}
-**提示:**如果您同时接收 Web 和电子邮件通知,您可以自动同步通知的已读或未读状态,以便在您阅读相应的电子邮件通知后,Web 通知自动标记为已读。 要启用此同步,您的电子邮件客户端必须能够查看来自 {% if currentVersion == "free-pro-team@latest" %}'`notifications@github.com`'{% else %}'网站管理员配置的无需回复电子邮件地址'{% endif %}的图像。
+**提示:**如果您同时接收 Web 和电子邮件通知,您可以自动同步通知的已读或未读状态,以便在您阅读相应的电子邮件通知后,Web 通知自动标记为已读。 To enable this sync, your email client must be able to view images from {% if currentVersion == "free-pro-team@latest" %}`notifications@github.com`{% else %}the `no-reply` email address {% if currentVersion == "github-ae@latest" %}for your {% data variables.product.product_name %} hostname{% elsif enterpriseServerVersions contains currentVersion %}for {% data variables.product.product_location %}, which your site administrator configures{% endif %}{% endif %}.
{% endtip %}
diff --git a/translations/zh-CN/data/reusables/profile/profile-readme.md b/translations/zh-CN/data/reusables/profile/profile-readme.md
index a00cff249858..630be86909f9 100644
--- a/translations/zh-CN/data/reusables/profile/profile-readme.md
+++ b/translations/zh-CN/data/reusables/profile/profile-readme.md
@@ -1 +1 @@
-如果将 README 文件添加到与用户名同名的公共仓库的根目录,则该 README 将自动显示在您的个人资料页面上。 您可以使用 GitHub Flavored Markdown 编辑您的个人资料以在您的个人资料 README,以在您的个人资料上创建个性化的区域。
+如果将 README 文件添加到与用户名同名的公共仓库的根目录,则该 README 将自动显示在您的个人资料页面上。 You can edit your profile README with {% data variables.product.company_short %} Flavored Markdown to create a personalized section on your profile. For more information, see "[Managing your profile README](/github/setting-up-and-managing-your-github-profile/managing-your-profile-readme)."
diff --git a/translations/zh-CN/data/reusables/repositories/administrators-can-disable-issues.md b/translations/zh-CN/data/reusables/repositories/administrators-can-disable-issues.md
new file mode 100644
index 000000000000..4352c32d5441
--- /dev/null
+++ b/translations/zh-CN/data/reusables/repositories/administrators-can-disable-issues.md
@@ -0,0 +1 @@
+Repository administrators can disable issues for a repository. 更多信息请参阅“[禁用议题](/github/managing-your-work-on-github/disabling-issues)”。
diff --git a/translations/zh-CN/data/reusables/repositories/desktop-fork.md b/translations/zh-CN/data/reusables/repositories/desktop-fork.md
index ae2f30e9a98d..d26ecea68a15 100644
--- a/translations/zh-CN/data/reusables/repositories/desktop-fork.md
+++ b/translations/zh-CN/data/reusables/repositories/desktop-fork.md
@@ -1 +1 @@
-您可以使用 {% data variables.product.prodname_desktop %} 复刻仓库。 更多信息请参阅“[从 {% data variables.product.prodname_desktop %} 克隆和复刻仓库](/desktop/contributing-to-projects/cloning-and-forking-repositories-from-github-desktop)”。
+您可以使用 {% data variables.product.prodname_desktop %} 复刻仓库。 For more information, see "[Cloning and forking repositories from {% data variables.product.prodname_desktop %}](/desktop/contributing-to-projects/cloning-and-forking-repositories-from-github-desktop)."
diff --git a/translations/zh-CN/data/reusables/repositories/you-can-fork.md b/translations/zh-CN/data/reusables/repositories/you-can-fork.md
index 7f499431c3ac..79505c5db733 100644
--- a/translations/zh-CN/data/reusables/repositories/you-can-fork.md
+++ b/translations/zh-CN/data/reusables/repositories/you-can-fork.md
@@ -1,3 +1,7 @@
-您可以将任何公共仓库复刻到您的用户帐户或任何您拥有仓库创建权限的组织。 更多信息请参阅“[组织的权限级别](/articles/permission-levels-for-an-organization)”。
+{% if currentVersion == "github-ae@latest" %}If the policies for your enterprise permit forking internal and private repositories, you{% else %}You{% endif %} can fork a repository to your user account or any organization where you have repository creation permissions. 更多信息请参阅“[组织的权限级别](/articles/permission-levels-for-an-organization)”。
-您可以将您具有访问权限的任何私有仓库复刻到您的用户帐户,以及 {% data variables.product.prodname_team %} 或 {% data variables.product.prodname_enterprise %} 上您拥有仓库创建权限的任何组织。 无法将私有仓库复刻到使用 {% data variables.product.prodname_free_team %} 的组织。{% if currentVersion == "free-pro-team@latest" %} 更多信息请参阅“[GitHub 的产品](/articles/githubs-products)”。{% endif %}
+{% if currentVersion == "free-pro-team@latest" or enterpriseServerVersions contains currentVersion %}
+
+If you have access to a private repository and the owner permits forking, you can fork the repository to your user account or any organization on {% if currentVersion == "free-pro-team@latest"%}{% data variables.product.prodname_team %}{% else %}{% data variables.product.product_location %}{% endif %} where you have repository creation permissions. {% if currentVersion == "free-pro-team@latest" %}You cannot fork a private repository to an organization using {% data variables.product.prodname_free_team %}. For more information, see "[GitHub's products](/articles/githubs-products)."{% endif %}
+
+{% endif %}
\ No newline at end of file
diff --git a/translations/zh-CN/data/reusables/search/required_login.md b/translations/zh-CN/data/reusables/search/required_login.md
index cbb406221521..6d8598a94a38 100644
--- a/translations/zh-CN/data/reusables/search/required_login.md
+++ b/translations/zh-CN/data/reusables/search/required_login.md
@@ -1 +1 @@
-必须登录才能跨所有公共仓库搜索代码。
+You must be signed into a user account on {% data variables.product.product_name %} to search for code across all public repositories.
diff --git a/translations/zh-CN/data/reusables/search/syntax_tips.md b/translations/zh-CN/data/reusables/search/syntax_tips.md
index 4cb4718cac0f..05e65d415ae9 100644
--- a/translations/zh-CN/data/reusables/search/syntax_tips.md
+++ b/translations/zh-CN/data/reusables/search/syntax_tips.md
@@ -1,7 +1,7 @@
{% tip %}
**Tips:**{% if enterpriseServerVersions contains currentVersion or currentVersion == "github-ae@latest" %}
- - 本文章包含在 {% data variables.product.prodname_dotcom %}.com 网站上的示例搜索,但您可以在 {% data variables.product.product_location %} 上使用相同的搜索过滤器。{% endif %}
+ - This article contains links to example searches on the {% data variables.product.prodname_dotcom_the_website %} website, but you can use the same search filters with {% data variables.product.product_name %}. In the linked example searches, replace `github.com` with the hostname for {% data variables.product.product_location %}.{% endif %}
- 有关可以添加到任何搜索限定符以进一步改善结果的搜索语法列表,请参阅“[了解搜索语法](/articles/understanding-the-search-syntax)”。
- 对多个字词的搜索词使用引号。 例如,如果要搜索具有标签 "In progress" 的议题,可搜索 `label:"in progress"`。 搜索不区分大小写。
diff --git a/translations/zh-CN/data/reusables/search/you-can-search-globally.md b/translations/zh-CN/data/reusables/search/you-can-search-globally.md
new file mode 100644
index 000000000000..f1e779352da2
--- /dev/null
+++ b/translations/zh-CN/data/reusables/search/you-can-search-globally.md
@@ -0,0 +1 @@
+您可以全局搜索所有 {% data variables.product.product_name %},也可搜索特定仓库或组织。
diff --git a/translations/zh-CN/data/reusables/ssh/add-ssh-key-to-ssh-agent-commandline.md b/translations/zh-CN/data/reusables/ssh/add-ssh-key-to-ssh-agent-commandline.md
index af4760bd1725..415f86736de3 100644
--- a/translations/zh-CN/data/reusables/ssh/add-ssh-key-to-ssh-agent-commandline.md
+++ b/translations/zh-CN/data/reusables/ssh/add-ssh-key-to-ssh-agent-commandline.md
@@ -1,3 +1,3 @@
```shell
-$ ssh-add ~/.ssh/id_rsa
+$ ssh-add ~/.ssh/id_ed25519
```
diff --git a/translations/zh-CN/data/reusables/ssh/add-ssh-key-to-ssh-agent.md b/translations/zh-CN/data/reusables/ssh/add-ssh-key-to-ssh-agent.md
index 04c01b634664..fb1ca5ec3428 100644
--- a/translations/zh-CN/data/reusables/ssh/add-ssh-key-to-ssh-agent.md
+++ b/translations/zh-CN/data/reusables/ssh/add-ssh-key-to-ssh-agent.md
@@ -1 +1 @@
-如果您创建了不同名称的密钥,或者您要添加不同名称的现有密钥,请将命令中的 *id_rsa* 替换为您的私钥文件的名称。
+If you created your key with a different name, or if you are adding an existing key that has a different name, replace *id_ed25519* in the command with the name of your private key file.
diff --git a/translations/zh-CN/data/variables/action_code_examples.yml b/translations/zh-CN/data/variables/action_code_examples.yml
index 84d3a4dfaa8b..9fe8643d564e 100644
--- a/translations/zh-CN/data/variables/action_code_examples.yml
+++ b/translations/zh-CN/data/variables/action_code_examples.yml
@@ -64,7 +64,7 @@
- publishing
-
title: GitHub Project Automation+
- description: Automate GitHub Project cards with any webhook event.
+ description: Automate GitHub Project cards with any webhook event
languages: JavaScript
href: alex-page/github-project-automation-plus
tags:
@@ -131,8 +131,7 @@
- publishing
-
title: Label your Pull Requests auto-magically (using committed files)
- description: >-
- Github action to label your pull requests auto-magically (using committed files)
+ description: Github action to label your pull requests auto-magically (using committed files)
languages: 'TypeScript, Dockerfile, JavaScript'
href: Decathlon/pull-request-labeler-action
tags:
@@ -147,3 +146,191 @@
tags:
- 拉取请求
- labels
+-
+ title: Get a list of file changes with a PR/Push
+ description: This action gives you will get outputs of the files that have changed in your repository
+ languages: 'TypeScript, Shell, JavaScript'
+ href: trilom/file-changes-action
+ tags:
+ - 工作流程
+ - 仓库
+-
+ title: Private actions in any workflow
+ description: Allows private GitHub Actions to be easily reused
+ languages: 'TypeScript, JavaScript, Shell'
+ href: InVisionApp/private-action-loader
+ tags:
+ - 工作流程
+ - tools
+-
+ title: Label your issues using the issue's contents
+ description: A GitHub Action to automatically tag issues with labels and assignees
+ languages: 'JavaScript, TypeScript'
+ href: damccorm/tag-ur-it
+ tags:
+ - 工作流程
+ - tools
+ - labels
+ - issues
+-
+ title: Rollback a GitHub Release
+ description: A GitHub Action to rollback or delete a release
+ languages: 'JavaScript'
+ href: author/action-rollback
+ tags:
+ - 工作流程
+ - 发行版
+-
+ title: Lock closed issues and Pull Requests
+ description: GitHub Action that locks closed issues and pull requests after a period of inactivity
+ languages: 'JavaScript'
+ href: dessant/lock-threads
+ tags:
+ - issues
+ - 拉取请求
+ - 工作流程
+-
+ title: Get Commit Difference Count Between Two Branches
+ description: This GitHub Action compares two branches and gives you the commit count between them
+ languages: 'JavaScript, Shell'
+ href: jessicalostinspace/commit-difference-action
+ tags:
+ - 提交
+ - 差异
+ - 工作流程
+-
+ title: Generate Release Notes Based on Git References
+ description: GitHub Action to generate changelogs, and release notes
+ languages: 'JavaScript, Shell'
+ href: metcalfc/changelog-generator
+ tags:
+ - cicd
+ - release-notes
+ - 工作流程
+ - 变更日志
+-
+ title: Enforce Policies on GitHub Repositories and Commits
+ description: Policy enforcement for your pipelines
+ languages: 'Go, Makefile, Dockerfile, Shell'
+ href: talos-systems/conform
+ tags:
+ - docker
+ - build-automation
+ - 工作流程
+-
+ title: Auto Label Issue Based
+ description: Automatically label an issue based on the issue description
+ languages: 'TypeScript, JavaScript, Dockerfile'
+ href: Renato66/auto-label
+ tags:
+ - labels
+ - 工作流程
+ - automation
+-
+ title: Update Configured GitHub Actions to the Latest Versions
+ description: CLI tool to check whehter all your actions are up-to-date or not
+ languages: 'C#, Inno Setup, PowerSHell, Shell'
+ href: fabasoad/ghacu
+ tags:
+ - versions
+ - cli
+ - 工作流程
+-
+ title: Create Issue Branch
+ description: GitHub Action that automates the creation of issue branches
+ languages: 'JavaScript, Shell'
+ href: robvanderleek/create-issue-branch
+ tags:
+ - probot
+ - issues
+ - labels
+-
+ title: Remove old artifacts
+ description: Customize artifact cleanup
+ languages: 'JavaScript, Shell'
+ href: c-hive/gha-remove-artifacts
+ tags:
+ - 构件
+ - 工作流程
+-
+ title: Sync Defined Files/Binaries to Wiki or External Repositories
+ description: GitHub Action to automatically sync changes to external repositories, like the wiki, for example
+ languages: 'Shell, Dockerfile'
+ href: kai-tub/external-repo-sync-action
+ tags:
+ - wiki
+ - sync
+ - 工作流程
+-
+ title: Create/Update/Delete a GitHub Wiki page based on any file
+ description: Updates your GitHub wiki by using rsync, allowing for exclusion of files and directories and actual deletion of files
+ languages: 'Shell, Dockerfile'
+ href: Andrew-Chen-Wang/github-wiki-action
+ tags:
+ - wiki
+ - docker
+ - 工作流程
+-
+ title: Prow GitHub Actions
+ description: Automation of policy enforcement, chat-ops, and automatic PR merging
+ languages: 'TypeScript, JavaScript'
+ href: jpmcb/prow-github-actions
+ tags:
+ - chat-ops
+ - prow
+ - 工作流程
+-
+ title: Check GitHub Status in your Workflow
+ description: Check GitHub Status in your workflow
+ languages: 'TypeScript, JavaScript'
+ href: crazy-max/ghaction-github-status
+ tags:
+ - 状态
+ - 监视
+ - 工作流程
+-
+ title: Manage labels on GitHub as code
+ description: GitHub Action to manage labels (create/rename/update/delete)
+ languages: 'TypeScript, JavaScript'
+ href: crazy-max/ghaction-github-labeler
+ tags:
+ - labels
+ - 工作流程
+ - automation
+-
+ title: Distribute funding in free and open source projects
+ description: Continuous Distribution of funding to project contributors and dependencies
+ languages: 'Python, Docerfile, Shell, Ruby'
+ href: protontypes/libreselery
+ tags:
+ - sponsors
+ - funding
+ - payment
+-
+ title: Herald rules for GitHub
+ description: Add reviewers, subscribers, labels and assignees to your PR
+ languages: 'TypeScript, JavaScript'
+ href: gagoar/use-herald-action
+ tags:
+ - reviewers
+ - labels
+ - assignees
+ - 拉取请求
+-
+ title: Codeowner validator
+ description: Ensures the correctness of your GitHub CODEOWNERS file, supports public and private GitHub repositories and also GitHub Enterprise installations
+ languages: 'Go, Shell, Makefile, Docerfile'
+ href: mszostok/codeowners-validator
+ tags:
+ - codeowners
+ - validate
+ - 工作流程
+-
+ title: Copybara Action
+ description: Move and transform code between repositories (ideal to maintain several repos from one monorepo)
+ languages: 'TypeScript, JavaScript, Shell'
+ href: olivr/copybara-action
+ tags:
+ - monorepo
+ - copybara
+ - 工作流程