diff --git a/.devcontainer/Dockerfile b/.devcontainer/Dockerfile
new file mode 100644
index 0000000000000..23c94155f3fd3
--- /dev/null
+++ b/.devcontainer/Dockerfile
@@ -0,0 +1,121 @@
+#-------------------------------------------------------------------------------------------------------------
+# Copyright (c) Microsoft Corporation. All rights reserved.
+# Licensed under the MIT License. See https://go.microsoft.com/fwlink/?linkid=2090316 for license information.
+#-------------------------------------------------------------------------------------------------------------
+
+FROM mcr.microsoft.com/vscode/devcontainers/typescript-node:0-12
+
+ARG TARGET_DISPLAY=":1"
+
+# VNC options
+ARG MAX_VNC_RESOLUTION=1920x1080x16
+ARG TARGET_VNC_RESOLUTION=1920x1080
+ARG TARGET_VNC_DPI=72
+ARG TARGET_VNC_PORT=5901
+ARG VNC_PASSWORD="vscode"
+
+# noVNC (VNC web client) options
+ARG INSTALL_NOVNC="true"
+ARG NOVNC_VERSION=1.1.0
+ARG TARGET_NOVNC_PORT=6080
+ARG WEBSOCKETIFY_VERSION=0.9.0
+
+# Firefox is useful for testing things like browser launch events, but optional
+ARG INSTALL_FIREFOX="false"
+
+# Expected non-root username from base image
+ARG USERNAME=node
+
+# Core environment variables for X11, VNC, and fluxbox
+ENV DBUS_SESSION_BUS_ADDRESS="autolaunch:" \
+ MAX_VNC_RESOLUTION="${MAX_VNC_RESOLUTION}" \
+ VNC_RESOLUTION="${TARGET_VNC_RESOLUTION}" \
+ VNC_DPI="${TARGET_VNC_DPI}" \
+ VNC_PORT="${TARGET_VNC_PORT}" \
+ NOVNC_PORT="${TARGET_NOVNC_PORT}" \
+ DISPLAY="${TARGET_DISPLAY}" \
+ LANG="en_US.UTF-8" \
+ LANGUAGE="en_US.UTF-8" \
+ VISUAL="nano" \
+ EDITOR="nano"
+
+# Configure apt and install packages
+RUN apt-get update \
+ && export DEBIAN_FRONTEND=noninteractive \
+ #
+ # Install the Cascadia Code fonts - https://github.com/microsoft/cascadia-code
+ && curl -sSL https://github.com/microsoft/cascadia-code/releases/download/v2004.30/CascadiaCode_2004.30.zip -o /tmp/cascadia-fonts.zip \
+ && unzip /tmp/cascadia-fonts.zip -d /tmp/cascadia-fonts \
+ && mkdir -p /usr/share/fonts/truetype/cascadia \
+ && mv /tmp/cascadia-fonts/ttf/* /usr/share/fonts/truetype/cascadia/ \
+ && rm -rf /tmp/cascadia-fonts.zip /tmp/cascadia-fonts \
+ #
+ # Install X11, fluxbox and VS Code dependencies
+ && apt-get -y install --no-install-recommends \
+ xvfb \
+ x11vnc \
+ fluxbox \
+ dbus-x11 \
+ x11-utils \
+ x11-xserver-utils \
+ xdg-utils \
+ fbautostart \
+ xterm \
+ eterm \
+ gnome-terminal \
+ gnome-keyring \
+ seahorse \
+ nautilus \
+ libx11-dev \
+ libxkbfile-dev \
+ libsecret-1-dev \
+ libnotify4 \
+ libnss3 \
+ libxss1 \
+ libasound2 \
+ xfonts-base \
+ xfonts-terminus \
+ fonts-noto \
+ fonts-wqy-microhei \
+ fonts-droid-fallback \
+ vim-tiny \
+ nano \
+ #
+ # [Optional] Install noVNC
+ && if [ "${INSTALL_NOVNC}" = "true" ]; then \
+ mkdir -p /usr/local/novnc \
+ && curl -sSL https://github.com/novnc/noVNC/archive/v${NOVNC_VERSION}.zip -o /tmp/novnc-install.zip \
+ && unzip /tmp/novnc-install.zip -d /usr/local/novnc \
+ && cp /usr/local/novnc/noVNC-${NOVNC_VERSION}/vnc_lite.html /usr/local/novnc/noVNC-${NOVNC_VERSION}/index.html \
+ && rm /tmp/novnc-install.zip \
+ && curl -sSL https://github.com/novnc/websockify/archive/v${WEBSOCKETIFY_VERSION}.zip -o /tmp/websockify-install.zip \
+ && unzip /tmp/websockify-install.zip -d /usr/local/novnc \
+ && apt-get -y install --no-install-recommends python-numpy \
+ && ln -s /usr/local/novnc/websockify-${WEBSOCKETIFY_VERSION} /usr/local/novnc/noVNC-${NOVNC_VERSION}/utils/websockify \
+ && rm /tmp/websockify-install.zip; \
+ fi \
+ #
+ # [Optional] Install Firefox
+ && if [ "${INSTALL_FIREFOX}" = "true" ]; then \
+ apt-get -y install --no-install-recommends firefox-esr; \
+ fi \
+ #
+ # Clean up
+ && apt-get autoremove -y \
+ && apt-get clean -y \
+ && rm -rf /var/lib/apt/lists/*
+
+COPY bin/init-dev-container.sh /usr/local/share/
+COPY bin/set-resolution /usr/local/bin/
+COPY fluxbox/* /root/.fluxbox/
+COPY fluxbox/* /home/${USERNAME}/.fluxbox/
+
+# Update privs, owners of config files
+RUN mkdir -p /var/run/dbus /root/.vnc /home/${USERNAME}/.vnc \
+ && touch /root/.Xmodmap /home/${USERNAME}/.Xmodmap \
+ && echo "${VNC_PASSWORD}" | tee /root/.vnc/passwd > /home/${USERNAME}/.vnc/passwd \
+ && chown -R ${USERNAME}:${USERNAME} /home/${USERNAME}/.Xmodmap /home/${USERNAME}/.fluxbox /home/${USERNAME}/.vnc \
+ && chmod +x /usr/local/share/init-dev-container.sh /usr/local/bin/set-resolution
+
+ENTRYPOINT ["/usr/local/share/init-dev-container.sh"]
+CMD ["sleep", "infinity"]
diff --git a/.devcontainer/README.md b/.devcontainer/README.md
new file mode 100644
index 0000000000000..e16795062d7ea
--- /dev/null
+++ b/.devcontainer/README.md
@@ -0,0 +1,82 @@
+# Code - OSS Development Container
+
+This repository includes configuration for a development container for working with Code - OSS in an isolated local container or using [Visual Studio Codespaces](https://aka.ms/vso).
+
+> **Tip:** The default VNC password is `vscode`. The VNC server runs on port `5901` with a web client at `6080`. For better performance, we recommend using a [VNC Viewer](https://www.realvnc.com/en/connect/download/viewer/). Applications like the macOS Screen Sharing app will not perform as well. [Chicken](https://sourceforge.net/projects/chicken/) is a good macOS alternative.
+
+## Quick start - local
+
+1. Install Docker Desktop or Docker for Linux on your local machine. (See [docs](https://aka.ms/vscode-remote/containers/getting-started) for additional details.)
+
+2. **Important**: Docker needs at least **4 Cores and 6 GB of RAM (8 GB recommended)** to run full build. If you on macOS, or using the old Hyper-V engine for Windows, update these values for Docker Desktop by right-clicking on the Docker status bar item, going to **Preferences/Settings > Resources > Advanced**.
+
+ > **Note:** The [Resource Monitor](https://marketplace.visualstudio.com/items?itemName=mutantdino.resourcemonitor) extension is included in the container so you can keep an eye on CPU/Memory in the status bar.
+
+3. Install [Visual Studio Code Stable](https://code.visualstudio.com/) or [Insiders](https://code.visualstudio.com/insiders/) and the [Remote - Containers](https://aka.ms/vscode-remote/download/containers) extension.
+
+ 
+
+ > Note that the Remote - Containers extension requires the Visual Studio Code distribution of Code - OSS. See the [FAQ](https://aka.ms/vscode-remote/faq/license) for details.
+
+4. Press Ctrl/Cmd + Shift + P and select **Remote - Containers: Open Repository in Container...**.
+
+ > **Tip:** While you can use your local source tree instead, operations like `yarn install` can be slow on macOS or using the Hyper-V engine on Windows. We recommend the "open repository" approach instead since it uses "named volume" rather than the local filesystem.
+
+5. Type `https://github.com/microsoft/vscode` (or a branch or PR URL) in the input box and press Enter.
+
+6. After the container is running, open a web browser and go to [http://localhost:6080](http://localhost:6080) or use a [VNC Viewer](https://www.realvnc.com/en/connect/download/viewer/) to connect to `localhost:5901` and enter `vscode` as the password.
+
+Anything you start in VS Code or the integrated terminal will appear here.
+
+Next: **[Try it out!](#try-it)**
+
+## Quick start - Codespaces
+
+>Note that the Codespaces browser-based editor cannot currently access the desktop environment in this container (due to a [missing feature](https://github.com/MicrosoftDocs/vsonline/issues/117)). We recommend using Visual Studio Code from the desktop to connect instead in the near term.
+
+1. Install [Visual Studio Code Stable](https://code.visualstudio.com/) or [Insiders](https://code.visualstudio.com/insiders/) and the [Visual Studio Codespaces](https://aka.ms/vscs-ext-vscode) extension.
+
+ 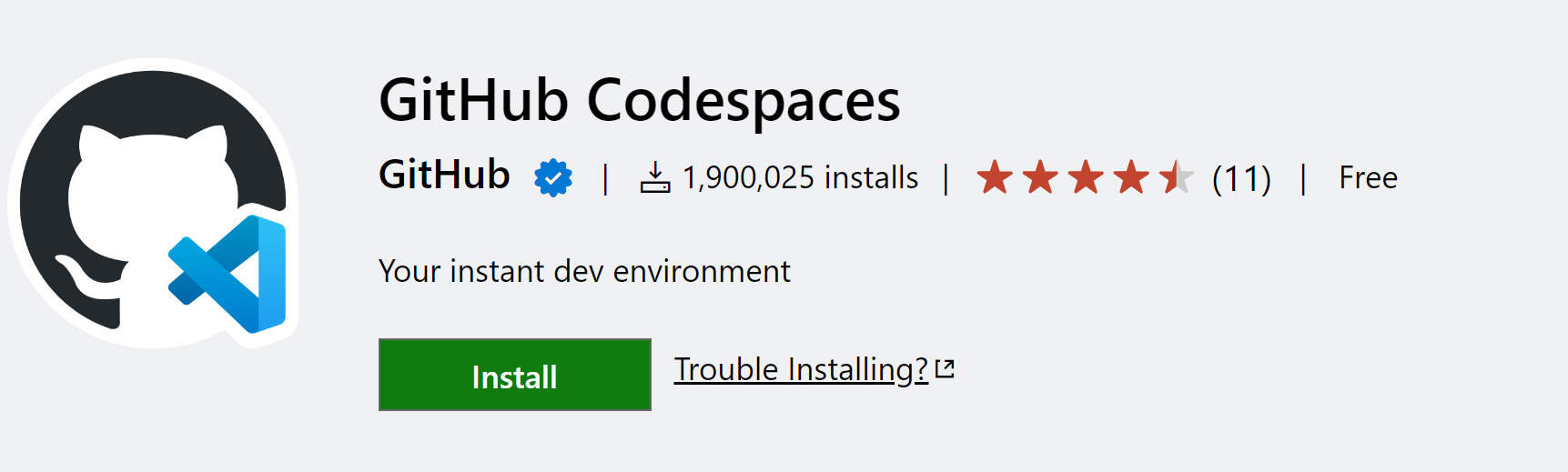
+
+ > Note that the Visual Studio Codespaces extension requires the Visual Studio Code distribution of Code - OSS.
+
+2. Sign in by pressing Ctrl/Cmd + Shift + P and selecting **Codespaces: Sign In**. You may also need to use the **Codespaces: Create Plan** if you do not have a plan. See the [Codespaces docs](https://aka.ms/vso-docs/vscode) for details.
+
+3. Press Ctrl/Cmd + Shift + P and select **Codespaces: Create New Codespace**.
+
+4. Use default settings (which should include **Standard** 4 core, 8 GB RAM Codespace), select a plan, and then enter the repository URL `https://github.com/microsoft/vscode` (or a branch or PR URL) in the input box when prompted.
+
+5. After the container is running, open a web browser and go to [http://localhost:6080](http://localhost:6080) or use a [VNC Viewer](https://www.realvnc.com/en/connect/download/viewer/) to connect to `localhost:5901` and enter `vscode` as the password.
+
+6. Anything you start in VS Code or the integrated terminal will appear here.
+
+## Try it!
+
+This container uses the [Fluxbox](http://fluxbox.org/) window manager to keep things lean. **Right-click on the desktop** to see menu options. It works with GNOME and GTK applications, so other tools can be installed if needed.
+
+Note you can also set the resolution from the command line by typing `set-resolution`.
+
+To start working with Code - OSS, follow these steps:
+
+1. In your local VS Code, open a terminal (Ctrl/Cmd + Shift + \`) and type the following commands:
+
+ ```bash
+ yarn install
+ bash scripts/code.sh
+ ```
+
+2. After the build is complete, open a web browser and go to [http://localhost:6080](http://localhost:6080) or use a [VNC Viewer](https://www.realvnc.com/en/connect/download/viewer/) to connect to `localhost:5901` and enter `vscode` as the password.
+
+3. You should now see Code - OSS!
+
+Next, let's try debugging.
+
+1. Shut down Code - OSS by clicking the box in the upper right corner of the Code - OSS window through your browser or VNC viewer.
+
+2. Go to your local VS Code client, and use Run / Debug view to launch the **VS Code** configuration. (Typically the default, so you can likely just press F5).
+
+ > **Note:** If launching times out, you can increase the value of `timeout` in the "VS Code", "Attach Main Process", "Attach Extension Host", and "Attach to Shared Process" configurations in [launch.json](../.vscode/launch.json). However, running `scripts/code.sh` first will set up Electron which will usually solve timeout issues.
+
+3. After a bit, Code - OSS will appear with the debugger attached!
+
+Enjoy!
diff --git a/.devcontainer/bin/init-dev-container.sh b/.devcontainer/bin/init-dev-container.sh
new file mode 100644
index 0000000000000..260cc27592243
--- /dev/null
+++ b/.devcontainer/bin/init-dev-container.sh
@@ -0,0 +1,91 @@
+#!/bin/bash
+
+NONROOT_USER=node
+LOG=/tmp/container-init.log
+
+# Execute the command it not already running
+startInBackgroundIfNotRunning()
+{
+ log "Starting $1."
+ echo -e "\n** $(date) **" | sudoIf tee -a /tmp/$1.log > /dev/null
+ if ! pidof $1 > /dev/null; then
+ keepRunningInBackground "$@"
+ while ! pidof $1 > /dev/null; do
+ sleep 1
+ done
+ log "$1 started."
+ else
+ echo "$1 is already running." | sudoIf tee -a /tmp/$1.log > /dev/null
+ log "$1 is already running."
+ fi
+}
+
+# Keep command running in background
+keepRunningInBackground()
+{
+ ($2 sh -c "while :; do echo [\$(date)] Process started.; $3; echo [\$(date)] Process exited!; sleep 5; done 2>&1" | sudoIf tee -a /tmp/$1.log > /dev/null & echo "$!" | sudoIf tee /tmp/$1.pid > /dev/null)
+}
+
+# Use sudo to run as root when required
+sudoIf()
+{
+ if [ "$(id -u)" -ne 0 ]; then
+ sudo "$@"
+ else
+ "$@"
+ fi
+}
+
+# Use sudo to run as non-root user if not already running
+sudoUserIf()
+{
+ if [ "$(id -u)" -eq 0 ]; then
+ sudo -u ${NONROOT_USER} "$@"
+ else
+ "$@"
+ fi
+}
+
+# Log messages
+log()
+{
+ echo -e "[$(date)] $@" | sudoIf tee -a $LOG > /dev/null
+}
+
+log "** SCRIPT START **"
+
+# Start dbus.
+log 'Running "/etc/init.d/dbus start".'
+if [ -f "/var/run/dbus/pid" ] && ! pidof dbus-daemon > /dev/null; then
+ sudoIf rm -f /var/run/dbus/pid
+fi
+sudoIf /etc/init.d/dbus start 2>&1 | sudoIf tee -a /tmp/dbus-daemon-system.log > /dev/null
+while ! pidof dbus-daemon > /dev/null; do
+ sleep 1
+done
+
+# Set up Xvfb.
+startInBackgroundIfNotRunning "Xvfb" sudoIf "Xvfb ${DISPLAY:-:1} +extension RANDR -screen 0 ${MAX_VNC_RESOLUTION:-1920x1080x16}"
+
+# Start fluxbox as a light weight window manager.
+startInBackgroundIfNotRunning "fluxbox" sudoUserIf "dbus-launch startfluxbox"
+
+# Start x11vnc
+startInBackgroundIfNotRunning "x11vnc" sudoIf "x11vnc -display ${DISPLAY:-:1} -rfbport ${VNC_PORT:-5901} -localhost -no6 -xkb -shared -forever -passwdfile $HOME/.vnc/passwd"
+
+# Set resolution
+/usr/local/bin/set-resolution ${VNC_RESOLUTION:-1280x720} ${VNC_DPI:-72}
+
+
+# Spin up noVNC if installed and not runnning.
+if [ -d "/usr/local/novnc" ] && [ "$(ps -ef | grep /usr/local/novnc/noVNC*/utils/launch.sh | grep -v grep)" = "" ]; then
+ keepRunningInBackground "noVNC" sudoIf "/usr/local/novnc/noVNC*/utils/launch.sh --listen ${NOVNC_PORT:-6080} --vnc localhost:${VNC_PORT:-5901}"
+ log "noVNC started."
+else
+ log "noVNC is already running or not installed."
+fi
+
+# Run whatever was passed in
+log "Executing \"$@\"."
+"$@"
+log "** SCRIPT EXIT **"
diff --git a/.devcontainer/bin/set-resolution b/.devcontainer/bin/set-resolution
new file mode 100644
index 0000000000000..5b4ca79f51883
--- /dev/null
+++ b/.devcontainer/bin/set-resolution
@@ -0,0 +1,25 @@
+#!/bin/bash
+RESOLUTION=${1:-${VNC_RESOLUTION:-1920x1080}}
+DPI=${2:-${VNC_DPI:-72}}
+if [ -z "$1" ]; then
+ echo -e "**Current Settings **\n"
+ xrandr
+ echo -n -e "\nEnter new resolution (WIDTHxHEIGHT, blank for ${RESOLUTION}, Ctrl+C to abort).\n> "
+ read NEW_RES
+ if [ "${NEW_RES}" != "" ]; then
+ RESOLUTION=${NEW_RES}
+ fi
+ if [ -z "$2" ]; then
+ echo -n -e "\nEnter new DPI (blank for ${DPI}, Ctrl+C to abort).\n> "
+ read NEW_DPI
+ if [ "${NEW_DPI}" != "" ]; then
+ DPI=${NEW_DPI}
+ fi
+ fi
+fi
+
+xrandr --fb ${RESOLUTION} --dpi ${DPI} > /dev/null 2>&1
+
+echo -e "\n**New Settings **\n"
+xrandr
+echo
diff --git a/.devcontainer/devcontainer.json b/.devcontainer/devcontainer.json
new file mode 100644
index 0000000000000..722bace6df74d
--- /dev/null
+++ b/.devcontainer/devcontainer.json
@@ -0,0 +1,45 @@
+{
+ "name": "Code - OSS",
+ "build": {
+ "dockerfile": "Dockerfile",
+ "args": {
+ "MAX_VNC_RESOLUTION": "1920x1080x16",
+ "TARGET_VNC_RESOLUTION": "1280x768",
+ "TARGET_VNC_PORT": "5901",
+ "TARGET_NOVNC_PORT": "6080",
+ "VNC_PASSWORD": "vscode",
+ "INSTALL_FIREFOX": "true"
+ }
+ },
+ "overrideCommand": false,
+ "runArgs": [
+ "--init",
+ // seccomp=unconfined is required for Chrome sandboxing
+ "--security-opt", "seccomp=unconfined"
+ ],
+
+ "settings": {
+ // zsh is also available
+ "terminal.integrated.shell.linux": "/bin/bash",
+ "resmon.show.battery": false,
+ "resmon.show.cpufreq": false,
+ "remote.extensionKind": {
+ "ms-vscode.js-debug-nightly": "workspace",
+ "msjsdiag.debugger-for-chrome": "workspace"
+ },
+ "debug.chrome.useV3": true
+ },
+
+ // noVNC, VNC ports
+ "forwardPorts": [6080, 5901],
+
+ "extensions": [
+ "dbaeumer.vscode-eslint",
+ "EditorConfig.EditorConfig",
+ "msjsdiag.debugger-for-chrome",
+ "mutantdino.resourcemonitor",
+ "GitHub.vscode-pull-request-github"
+ ],
+
+ "remoteUser": "node"
+}
diff --git a/.devcontainer/fluxbox/apps b/.devcontainer/fluxbox/apps
new file mode 100644
index 0000000000000..d43f05e9e2057
--- /dev/null
+++ b/.devcontainer/fluxbox/apps
@@ -0,0 +1,9 @@
+[app] (name=code-oss-dev)
+ [Position] (CENTER) {0 0}
+ [Maximized] {yes}
+ [Dimensions] {100% 100%}
+[end]
+[transient] (role=GtkFileChooserDialog)
+ [Position] (CENTER) {0 0}
+ [Dimensions] {70% 70%}
+[end]
diff --git a/.devcontainer/fluxbox/init b/.devcontainer/fluxbox/init
new file mode 100644
index 0000000000000..a6b8d73fa73d4
--- /dev/null
+++ b/.devcontainer/fluxbox/init
@@ -0,0 +1,9 @@
+session.menuFile: ~/.fluxbox/menu
+session.keyFile: ~/.fluxbox/keys
+session.styleFile: /usr/share/fluxbox/styles//Squared_for_Debian
+session.configVersion: 13
+session.screen0.workspaces: 1
+session.screen0.workspacewarping: false
+session.screen0.toolbar.widthPercent: 100
+session.screen0.strftimeFormat: %d %b, %a %02k:%M:%S
+session.screen0.toolbar.tools: prevworkspace, workspacename, nextworkspace, clock, prevwindow, nextwindow, iconbar, systemtray
diff --git a/.devcontainer/fluxbox/menu b/.devcontainer/fluxbox/menu
new file mode 100644
index 0000000000000..ff5955a2fe75a
--- /dev/null
+++ b/.devcontainer/fluxbox/menu
@@ -0,0 +1,16 @@
+[begin] ( Code - OSS Development Container )
+ [exec] (File Manager) { nautilus ~ } <>
+ [exec] (Terminal) {/usr/bin/gnome-terminal --working-directory=~ } <>
+ [exec] (Start Code - OSS) { x-terminal-emulator -T "Code - OSS Build" -e bash /workspaces/vscode*/scripts/code.sh } <>
+ [submenu] (System >) {}
+ [exec] (Set Resolution) { x-terminal-emulator -T "Set Resolution" -e bash /usr/local/bin/set-resolution } <>
+ [exec] (Passwords and Keys) { seahorse } <>
+ [exec] (Top) { x-terminal-emulator -T "Top" -e /usr/bin/top } <>
+ [exec] (Editres) {editres} <>
+ [exec] (Xfontsel) {xfontsel} <>
+ [exec] (Xkill) {xkill} <>
+ [exec] (Xrefresh) {xrefresh} <>
+ [end]
+ [config] (Configuration >)
+ [workspaces] (Workspaces >)
+[end]
diff --git a/.eslintignore b/.eslintignore
new file mode 100644
index 0000000000000..f186c7ecd78d8
--- /dev/null
+++ b/.eslintignore
@@ -0,0 +1,15 @@
+**/vs/nls.build.js
+**/vs/nls.js
+**/vs/css.build.js
+**/vs/css.js
+**/vs/loader.js
+**/insane/**
+**/marked/**
+**/test/**/*.js
+**/node_modules/**
+**/vscode-api-tests/testWorkspace/**
+**/vscode-api-tests/testWorkspace2/**
+**/extensions/**/out/**
+**/extensions/**/build/**
+**/extensions/markdown-language-features/media/**
+**/extensions/typescript-basics/test/colorize-fixtures/**
diff --git a/.eslintrc.json b/.eslintrc.json
index 29efa7cbbc202..df3c5ad560c4b 100644
--- a/.eslintrc.json
+++ b/.eslintrc.json
@@ -1,20 +1,994 @@
{
- "root": true,
- "env": {
- "node": true,
- "es6": true
- },
- "rules": {
- "no-console": 0,
- "no-cond-assign": 0,
- "no-unused-vars": 1,
- "no-extra-semi": "warn",
- "semi": "warn"
- },
- "extends": "eslint:recommended",
- "parserOptions": {
- "ecmaFeatures": {
- "experimentalObjectRestSpread": true
- }
- }
+ "root": true,
+ "parser": "@typescript-eslint/parser",
+ "parserOptions": {
+ "ecmaVersion": 6,
+ "sourceType": "module"
+ },
+ "plugins": [
+ "@typescript-eslint",
+ "jsdoc"
+ ],
+ "rules": {
+ "constructor-super": "warn",
+ "curly": "warn",
+ "eqeqeq": "warn",
+ "no-buffer-constructor": "warn",
+ "no-caller": "warn",
+ "no-debugger": "warn",
+ "no-duplicate-case": "warn",
+ "no-duplicate-imports": "warn",
+ "no-eval": "warn",
+ "no-extra-semi": "warn",
+ "no-new-wrappers": "warn",
+ "no-redeclare": "off",
+ "no-sparse-arrays": "warn",
+ "no-throw-literal": "warn",
+ "no-unsafe-finally": "warn",
+ "no-unused-labels": "warn",
+ "no-restricted-globals": [
+ "warn",
+ "name",
+ "length",
+ "event",
+ "closed",
+ "external",
+ "status",
+ "origin",
+ "orientation",
+ "context"
+ ], // non-complete list of globals that are easy to access unintentionally
+ "no-var": "warn",
+ "jsdoc/no-types": "warn",
+ "semi": "off",
+ "@typescript-eslint/semi": "warn",
+ "@typescript-eslint/naming-convention": [
+ "warn",
+ {
+ "selector": "class",
+ "format": [
+ "PascalCase"
+ ]
+ }
+ ],
+ "code-no-unused-expressions": [
+ "warn",
+ {
+ "allowTernary": true
+ }
+ ],
+ "code-translation-remind": "warn",
+ "code-no-nls-in-standalone-editor": "warn",
+ "code-no-standalone-editor": "warn",
+ "code-no-unexternalized-strings": "warn",
+ "code-layering": [
+ "warn",
+ {
+ "common": [],
+ "node": [
+ "common"
+ ],
+ "browser": [
+ "common"
+ ],
+ "electron-sandbox": [
+ "common",
+ "browser"
+ ],
+ "electron-browser": [
+ "common",
+ "browser",
+ "node",
+ "electron-sandbox"
+ ],
+ "electron-main": [
+ "common",
+ "node"
+ ]
+ }
+ ],
+ "code-import-patterns": [
+ "warn",
+ // !!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!
+ // !!! Do not relax these rules !!!
+ // !!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!
+ {
+ "target": "**/vs/base/common/**",
+ "restrictions": [
+ "vs/nls",
+ "**/vs/base/common/**"
+ ]
+ },
+ {
+ "target": "**/vs/base/test/common/**",
+ "restrictions": [
+ "assert",
+ "sinon",
+ "vs/nls",
+ "**/vs/base/common/**",
+ "**/vs/base/test/common/**"
+ ]
+ },
+ {
+ "target": "**/vs/base/browser/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/{common,browser}/**"
+ ]
+ },
+ {
+ "target": "**/vs/base/electron-sandbox/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/{common,browser,electron-sandbox}/**"
+ ]
+ },
+ {
+ "target": "**/vs/base/node/**",
+ "restrictions": [
+ "vs/nls",
+ "**/vs/base/{common,node}/**",
+ "*" // node modules
+ ]
+ },
+ {
+ // vs/base/test/browser contains tests for vs/base/browser
+ "target": "**/vs/base/test/browser/**",
+ "restrictions": [
+ "assert",
+ "sinon",
+ "vs/nls",
+ "**/vs/base/{common,browser}/**",
+ "**/vs/base/test/{common,browser}/**"
+ ]
+ },
+ {
+ "target": "**/vs/base/parts/*/common/**",
+ "restrictions": [
+ "vs/nls",
+ "**/vs/base/common/**",
+ "**/vs/base/parts/*/common/**"
+ ]
+ },
+ {
+ "target": "**/vs/base/parts/*/browser/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/{common,browser}/**",
+ "**/vs/base/parts/*/{common,browser}/**"
+ ]
+ },
+ {
+ "target": "**/vs/base/parts/*/node/**",
+ "restrictions": [
+ "vs/nls",
+ "**/vs/base/{common,node}/**",
+ "**/vs/base/parts/*/{common,node}/**",
+ "*" // node modules
+ ]
+ },
+ {
+ "target": "**/vs/base/parts/*/electron-sandbox/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/{common,browser,electron-sandbox}/**",
+ "**/vs/base/parts/*/{common,browser,electron-sandbox}/**"
+ ]
+ },
+ {
+ "target": "**/vs/base/parts/*/electron-browser/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/base/parts/*/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "*" // node modules
+ ]
+ },
+ {
+ "target": "**/vs/base/parts/*/electron-main/**",
+ "restrictions": [
+ "vs/nls",
+ "**/vs/base/{common,node,electron-main}/**",
+ "**/vs/base/parts/*/{common,node,electron-main}/**",
+ "*" // node modules
+ ]
+ },
+ {
+ "target": "**/vs/platform/*/common/**",
+ "restrictions": [
+ "vs/nls",
+ "**/vs/base/common/**",
+ "**/vs/base/parts/*/common/**",
+ "**/vs/platform/*/common/**"
+ ]
+ },
+ {
+ "target": "**/vs/platform/*/test/common/**",
+ "restrictions": [
+ "assert",
+ "sinon",
+ "vs/nls",
+ "**/vs/base/common/**",
+ "**/vs/base/parts/*/common/**",
+ "**/vs/base/test/common/**",
+ "**/vs/platform/*/common/**",
+ "**/vs/platform/*/test/common/**"
+ ]
+ },
+ {
+ "target": "**/vs/platform/*/browser/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/{common,browser}/**",
+ "**/vs/base/parts/*/{common,browser}/**",
+ "**/vs/platform/*/{common,browser}/**"
+ ]
+ },
+ {
+ "target": "**/vs/platform/*/node/**",
+ "restrictions": [
+ "vs/nls",
+ "**/vs/base/{common,node}/**",
+ "**/vs/base/parts/*/{common,node}/**",
+ "**/vs/platform/*/{common,node}/**",
+ "*" // node modules
+ ]
+ },
+ {
+ "target": "**/vs/platform/*/electron-sandbox/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/{common,browser,electron-sandbox}/**",
+ "**/vs/base/parts/*/{common,browser,electron-sandbox}/**",
+ "**/vs/platform/*/{common,browser,electron-sandbox}/**"
+ ]
+ },
+ {
+ "target": "**/vs/platform/*/electron-browser/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/base/parts/*/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/platform/*/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "*" // node modules
+ ]
+ },
+ {
+ "target": "**/vs/platform/*/electron-main/**",
+ "restrictions": [
+ "vs/nls",
+ "**/vs/base/{common,node,electron-main}/**",
+ "**/vs/base/parts/*/{common,node,electron-main}/**",
+ "**/vs/platform/*/{common,node,electron-main}/**",
+ "**/vs/code/**",
+ "*" // node modules
+ ]
+ },
+ {
+ "target": "**/vs/platform/*/test/browser/**",
+ "restrictions": [
+ "assert",
+ "sinon",
+ "vs/nls",
+ "**/vs/base/{common,browser}/**",
+ "**/vs/platform/*/{common,browser}/**",
+ "**/vs/platform/*/test/{common,browser}/**"
+ ]
+ },
+ {
+ "target": "**/vs/editor/common/**",
+ "restrictions": [
+ "vs/nls",
+ "**/vs/base/common/**",
+ "**/vs/base/worker/**",
+ "**/vs/platform/*/common/**",
+ "**/vs/editor/common/**"
+ ]
+ },
+ {
+ "target": "**/vs/editor/test/common/**",
+ "restrictions": [
+ "assert",
+ "sinon",
+ "vs/nls",
+ "**/vs/base/common/**",
+ "**/vs/platform/*/common/**",
+ "**/vs/platform/*/test/common/**",
+ "**/vs/editor/common/**",
+ "**/vs/editor/test/common/**"
+ ]
+ },
+ {
+ "target": "**/vs/editor/browser/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/{common,browser}/**",
+ "**/vs/platform/*/{common,browser}/**",
+ "**/vs/editor/{common,browser}/**"
+ ]
+ },
+ {
+ "target": "**/vs/editor/test/browser/**",
+ "restrictions": [
+ "assert",
+ "sinon",
+ "vs/nls",
+ "**/vs/base/{common,browser}/**",
+ "**/vs/platform/*/{common,browser}/**",
+ "**/vs/platform/*/test/{common,browser}/**",
+ "**/vs/editor/{common,browser}/**",
+ "**/vs/editor/test/{common,browser}/**"
+ ]
+ },
+ {
+ "target": "**/vs/editor/standalone/common/**",
+ "restrictions": [
+ "vs/nls",
+ "**/vs/base/common/**",
+ "**/vs/platform/*/common/**",
+ "**/vs/editor/common/**",
+ "**/vs/editor/standalone/common/**"
+ ]
+ },
+ {
+ "target": "**/vs/editor/standalone/test/common/**",
+ "restrictions": [
+ "assert",
+ "sinon",
+ "vs/nls",
+ "**/vs/base/common/**",
+ "**/vs/platform/*/common/**",
+ "**/vs/platform/*/test/common/**",
+ "**/vs/editor/common/**",
+ "**/vs/editor/test/common/**"
+ ]
+ },
+ {
+ "target": "**/vs/editor/standalone/browser/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/{common,browser}/**",
+ "**/vs/base/parts/*/{common,browser}/**",
+ "**/vs/platform/*/{common,browser}/**",
+ "**/vs/editor/{common,browser}/**",
+ "**/vs/editor/contrib/**",
+ "**/vs/editor/standalone/{common,browser}/**"
+ ]
+ },
+ {
+ "target": "**/vs/editor/standalone/test/browser/**",
+ "restrictions": [
+ "assert",
+ "sinon",
+ "vs/nls",
+ "**/vs/base/{common,browser}/**",
+ "**/vs/platform/*/{common,browser}/**",
+ "**/vs/platform/*/test/{common,browser}/**",
+ "**/vs/editor/{common,browser}/**",
+ "**/vs/editor/standalone/{common,browser}/**",
+ "**/vs/editor/test/{common,browser}/**"
+ ]
+ },
+ {
+ "target": "**/vs/editor/contrib/*/test/**",
+ "restrictions": [
+ "assert",
+ "sinon",
+ "vs/nls",
+ "**/vs/base/{common,browser}/**",
+ "**/vs/base/test/{common,browser}/**",
+ "**/vs/base/parts/*/{common,browser}/**",
+ "**/vs/platform/*/{common,browser}/**",
+ "**/vs/platform/*/test/{common,browser}/**",
+ "**/vs/editor/{common,browser}/**",
+ "**/vs/editor/test/{common,browser}/**",
+ "**/vs/editor/contrib/**"
+ ]
+ },
+ {
+ "target": "**/vs/editor/contrib/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/{common,browser}/**",
+ "**/vs/base/parts/*/{common,browser}/**",
+ "**/vs/platform/{common,browser}/**",
+ "**/vs/platform/*/{common,browser}/**",
+ "**/vs/editor/{common,browser}/**",
+ "**/vs/editor/contrib/**"
+ ]
+ },
+ {
+ "target": "**/vs/workbench/common/**",
+ "restrictions": [
+ "vs/nls",
+ "**/vs/base/common/**",
+ "**/vs/base/parts/*/common/**",
+ "**/vs/platform/*/common/**",
+ "**/vs/editor/common/**",
+ "**/vs/editor/contrib/*/common/**",
+ "**/vs/workbench/common/**",
+ "**/vs/workbench/services/*/common/**",
+ "assert"
+ ]
+ },
+ {
+ "target": "**/vs/workbench/browser/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/{common,browser}/**",
+ "**/vs/base/parts/*/{common,browser}/**",
+ "**/vs/platform/*/{common,browser}/**",
+ "**/vs/editor/{common,browser}/**",
+ "**/vs/editor/contrib/**", // editor/contrib is equivalent to /browser/ by convention
+ "**/vs/workbench/workbench.web.api",
+ "**/vs/workbench/{common,browser}/**",
+ "**/vs/workbench/services/*/{common,browser}/**",
+ "assert"
+ ]
+ },
+ {
+ "target": "**/vs/workbench/api/common/**",
+ "restrictions": [
+ "vscode",
+ "vs/nls",
+ "**/vs/base/common/**",
+ "**/vs/platform/*/common/**",
+ "**/vs/editor/common/**",
+ "**/vs/editor/contrib/*/common/**",
+ "**/vs/workbench/api/common/**",
+ "**/vs/workbench/common/**",
+ "**/vs/workbench/services/*/common/**",
+ "**/vs/workbench/contrib/*/common/**"
+ ]
+ },
+ {
+ "target": "**/vs/workbench/api/worker/**",
+ "restrictions": [
+ "vscode",
+ "vs/nls",
+ "**/vs/**/{common,worker}/**"
+ ]
+ },
+ {
+ "target": "**/vs/workbench/electron-sandbox/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/{common,browser,electron-sandbox}/**",
+ "**/vs/base/parts/*/{common,browser,electron-sandbox}/**",
+ "**/vs/platform/*/{common,browser,electron-sandbox}/**",
+ "**/vs/editor/{common,browser,electron-sandbox}/**",
+ "**/vs/editor/contrib/**", // editor/contrib is equivalent to /browser/ by convention
+ "**/vs/workbench/{common,browser,electron-sandbox}/**",
+ "**/vs/workbench/api/{common,browser,electron-sandbox}/**",
+ "**/vs/workbench/services/*/{common,browser,electron-sandbox}/**"
+ ]
+ },
+ {
+ "target": "**/vs/workbench/electron-browser/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/base/parts/*/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/platform/*/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/editor/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/editor/contrib/**", // editor/contrib is equivalent to /browser/ by convention
+ "**/vs/workbench/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/workbench/api/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/workbench/services/*/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "*" // node modules
+ ]
+ },
+ {
+ "target": "**/vs/workbench/services/**/test/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/**",
+ "**/vs/platform/**",
+ "**/vs/editor/**",
+ "**/vs/workbench/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "vs/workbench/contrib/files/common/editors/fileEditorInput",
+ "**/vs/workbench/services/**",
+ "**/vs/workbench/test/**",
+ "*" // node modules
+ ]
+ },
+ {
+ "target": "**/vs/workbench/services/**/common/**",
+ "restrictions": [
+ "vs/nls",
+ "**/vs/base/**/common/**",
+ "**/vs/platform/**/common/**",
+ "**/vs/editor/common/**",
+ "**/vs/workbench/workbench.web.api",
+ "**/vs/workbench/common/**",
+ "**/vs/workbench/services/**/common/**",
+ "**/vs/workbench/api/**/common/**",
+ "vscode-textmate",
+ "vscode-oniguruma",
+ "iconv-lite-umd",
+ "semver-umd"
+ ]
+ },
+ {
+ "target": "**/vs/workbench/services/**/worker/**",
+ "restrictions": [
+ "vs/nls",
+ "**/vs/base/**/common/**",
+ "**/vs/platform/**/common/**",
+ "**/vs/editor/common/**",
+ "**/vs/workbench/**/common/**",
+ "**/vs/workbench/**/worker/**",
+ "**/vs/workbench/services/**/common/**",
+ "vscode"
+ ]
+ },
+ {
+ "target": "**/vs/workbench/services/**/browser/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/**/{common,browser,worker}/**",
+ "**/vs/platform/**/{common,browser}/**",
+ "**/vs/editor/{common,browser}/**",
+ "**/vs/workbench/workbench.web.api",
+ "**/vs/workbench/{common,browser}/**",
+ "**/vs/workbench/api/{common,browser}/**",
+ "**/vs/workbench/services/**/{common,browser}/**",
+ "vscode-textmate",
+ "vscode-oniguruma"
+ ]
+ },
+ {
+ "target": "**/vs/workbench/services/**/node/**",
+ "restrictions": [
+ "vs/nls",
+ "**/vs/base/**/{common,node}/**",
+ "**/vs/platform/**/{common,node}/**",
+ "**/vs/editor/{common,node}/**",
+ "**/vs/workbench/{common,node}/**",
+ "**/vs/workbench/api/{common,node}/**",
+ "**/vs/workbench/services/**/{common,node}/**",
+ "*" // node modules
+ ]
+ },
+ {
+ "target": "**/vs/workbench/services/**/electron-sandbox/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/**/{common,browser,worker,electron-sandbox}/**",
+ "**/vs/platform/**/{common,browser,electron-sandbox}/**",
+ "**/vs/editor/**",
+ "**/vs/workbench/{common,browser,electron-sandbox}/**",
+ "**/vs/workbench/api/{common,browser,electron-sandbox}/**",
+ "**/vs/workbench/services/**/{common,browser,electron-sandbox}/**"
+ ]
+ },
+ {
+ "target": "**/vs/workbench/services/**/electron-browser/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/**/{common,browser,worker,node,electron-sandbox,electron-browser}/**",
+ "**/vs/platform/**/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/editor/**",
+ "**/vs/workbench/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/workbench/api/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/workbench/services/**/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "*" // node modules
+ ]
+ },
+ {
+ "target": "**/vs/workbench/contrib/**/test/**",
+ "restrictions": [
+ "assert",
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/**",
+ "**/vs/platform/**",
+ "**/vs/editor/**",
+ "**/vs/workbench/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/workbench/services/**",
+ "**/vs/workbench/contrib/**",
+ "**/vs/workbench/test/**",
+ "*"
+ ]
+ },
+ {
+ "target": "**/vs/workbench/contrib/terminal/browser/**",
+ "restrictions": [
+ // xterm and its addons are strictly browser-only components
+ "xterm",
+ "xterm-addon-*",
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/**/{common,browser}/**",
+ "**/vs/platform/**/{common,browser}/**",
+ "**/vs/editor/**",
+ "**/vs/workbench/{common,browser}/**",
+ "**/vs/workbench/contrib/**/{common,browser}/**",
+ "**/vs/workbench/services/**/{common,browser}/**"
+ ]
+ },
+ {
+ "target": "**/vs/workbench/contrib/extensions/browser/**",
+ "restrictions": [
+ "semver-umd",
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/**/{common,browser}/**",
+ "**/vs/platform/**/{common,browser}/**",
+ "**/vs/editor/**",
+ "**/vs/workbench/{common,browser}/**",
+ "**/vs/workbench/contrib/**/{common,browser}/**",
+ "**/vs/workbench/services/**/{common,browser}/**"
+ ]
+ },
+ {
+ "target": "**/vs/workbench/contrib/update/browser/update.ts",
+ "restrictions": [
+ "semver-umd",
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/**/{common,browser}/**",
+ "**/vs/platform/**/{common,browser}/**",
+ "**/vs/editor/**",
+ "**/vs/workbench/{common,browser}/**",
+ "**/vs/workbench/contrib/**/{common,browser}/**",
+ "**/vs/workbench/services/**/{common,browser}/**"
+ ]
+ },
+ {
+ "target": "**/vs/workbench/contrib/notebook/common/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/**/{common,worker}/**",
+ "**/vs/platform/**/common/**",
+ "**/vs/editor/**",
+ "**/vs/workbench/common/**",
+ "**/vs/workbench/api/common/**",
+ "**/vs/workbench/services/**/common/**",
+ "**/vs/workbench/contrib/**/common/**"
+ ]
+ },
+ {
+ "target": "**/vs/workbench/contrib/**/common/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/**/common/**",
+ "**/vs/platform/**/common/**",
+ "**/vs/editor/**",
+ "**/vs/workbench/common/**",
+ "**/vs/workbench/api/common/**",
+ "**/vs/workbench/services/**/common/**",
+ "**/vs/workbench/contrib/**/common/**"
+ ]
+ },
+ {
+ "target": "**/vs/workbench/contrib/**/browser/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/**/{common,browser}/**",
+ "**/vs/platform/**/{common,browser}/**",
+ "**/vs/editor/**",
+ "**/vs/workbench/{common,browser}/**",
+ "**/vs/workbench/api/{common,browser}/**",
+ "**/vs/workbench/services/**/{common,browser}/**",
+ "**/vs/workbench/contrib/**/{common,browser}/**"
+ ]
+ },
+ {
+ "target": "**/vs/workbench/contrib/**/node/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/**/{common,node}/**",
+ "**/vs/platform/**/{common,node}/**",
+ "**/vs/editor/**/common/**",
+ "**/vs/workbench/{common,node}/**",
+ "**/vs/workbench/api/{common,node}/**",
+ "**/vs/workbench/services/**/{common,node}/**",
+ "**/vs/workbench/contrib/**/{common,node}/**",
+ "*" // node modules
+ ]
+ },
+ {
+ "target": "**/vs/workbench/contrib/**/electron-sandbox/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/**/{common,browser,worker,electron-sandbox}/**",
+ "**/vs/platform/**/{common,browser,electron-sandbox}/**",
+ "**/vs/editor/**",
+ "**/vs/workbench/{common,browser,electron-sandbox}/**",
+ "**/vs/workbench/api/{common,browser,electron-sandbox}/**",
+ "**/vs/workbench/services/**/{common,browser,electron-sandbox}/**",
+ "**/vs/workbench/contrib/**/{common,browser,electron-sandbox}/**"
+ ]
+ },
+ {
+ "target": "**/vs/workbench/contrib/**/electron-browser/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/**/{common,browser,worker,node,electron-sandbox,electron-browser}/**",
+ "**/vs/platform/**/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/editor/**",
+ "**/vs/workbench/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/workbench/api/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/workbench/services/**/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/workbench/contrib/**/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "*" // node modules
+ ]
+ },
+ {
+ "target": "**/vs/code/browser/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/**/{common,browser}/**",
+ "**/vs/base/parts/**/{common,browser}/**",
+ "**/vs/platform/**/{common,browser}/**",
+ "**/vs/code/**/{common,browser}/**",
+ "**/vs/workbench/workbench.web.api"
+ ]
+ },
+ {
+ "target": "**/vs/code/node/**",
+ "restrictions": [
+ "vs/nls",
+ "**/vs/base/**/{common,node}/**",
+ "**/vs/base/parts/**/{common,node}/**",
+ "**/vs/platform/**/{common,node}/**",
+ "**/vs/code/**/{common,node}/**",
+ "*" // node modules
+ ]
+ },
+ {
+ "target": "**/vs/code/electron-browser/**",
+ "restrictions": [
+ "vs/nls",
+ "vs/css!./**/*",
+ "**/vs/base/**/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/base/parts/**/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/platform/**/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/code/**/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "*" // node modules
+ ]
+ },
+ {
+ "target": "**/vs/code/electron-main/**",
+ "restrictions": [
+ "vs/nls",
+ "**/vs/base/**/{common,node,electron-main}/**",
+ "**/vs/base/parts/**/{common,node,electron-main}/**",
+ "**/vs/platform/**/{common,node,electron-main}/**",
+ "**/vs/code/**/{common,node,electron-main}/**",
+ "*" // node modules
+ ]
+ },
+ {
+ "target": "**/vs/server/**",
+ "restrictions": [
+ "vs/nls",
+ "**/vs/base/**/{common,node}/**",
+ "**/vs/base/parts/**/{common,node}/**",
+ "**/vs/platform/**/{common,node}/**",
+ "**/vs/workbench/**/{common,node}/**",
+ "**/vs/server/**",
+ "**/vs/code/**/{common,node}/**",
+ "*" // node modules
+ ]
+ },
+ {
+ "target": "**/src/vs/workbench/workbench.common.main.ts",
+ "restrictions": [
+ "vs/nls",
+ "**/vs/base/**/{common,browser}/**",
+ "**/vs/base/parts/**/{common,browser}/**",
+ "**/vs/platform/**/{common,browser}/**",
+ "**/vs/editor/**",
+ "**/vs/workbench/**/{common,browser}/**"
+ ]
+ },
+ {
+ "target": "**/src/vs/workbench/workbench.web.main.ts",
+ "restrictions": [
+ "vs/nls",
+ "**/vs/base/**/{common,browser}/**",
+ "**/vs/base/parts/**/{common,browser}/**",
+ "**/vs/platform/**/{common,browser}/**",
+ "**/vs/editor/**",
+ "**/vs/workbench/**/{common,browser}/**",
+ "**/vs/workbench/workbench.common.main"
+ ]
+ },
+ {
+ "target": "**/src/vs/workbench/workbench.web.api.ts",
+ "restrictions": [
+ "vs/nls",
+ "**/vs/base/**/{common,browser}/**",
+ "**/vs/base/parts/**/{common,browser}/**",
+ "**/vs/platform/**/{common,browser}/**",
+ "**/vs/editor/**",
+ "**/vs/workbench/**/{common,browser}/**",
+ "**/vs/workbench/workbench.web.main"
+ ]
+ },
+ {
+ "target": "**/src/vs/workbench/workbench.sandbox.main.ts",
+ "restrictions": [
+ "vs/nls",
+ "**/vs/base/**/{common,browser,electron-sandbox}/**",
+ "**/vs/base/parts/**/{common,browser,electron-sandbox}/**",
+ "**/vs/platform/**/{common,browser,electron-sandbox}/**",
+ "**/vs/editor/**",
+ "**/vs/workbench/**/{common,browser,electron-sandbox}/**",
+ "**/vs/workbench/workbench.common.main"
+ ]
+ },
+ {
+ "target": "**/src/vs/workbench/workbench.desktop.main.ts",
+ "restrictions": [
+ "vs/nls",
+ "**/vs/base/**/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/base/parts/**/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/platform/**/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/editor/**",
+ "**/vs/workbench/**/{common,browser,node,electron-sandbox,electron-browser}/**",
+ "**/vs/workbench/workbench.common.main",
+ "**/vs/workbench/workbench.sandbox.main"
+ ]
+ },
+ {
+ "target": "**/extensions/**",
+ "restrictions": "**/*"
+ },
+ {
+ "target": "**/test/smoke/**",
+ "restrictions": [
+ "**/test/smoke/**",
+ "*" // node modules
+ ]
+ },
+ {
+ "target": "**/test/automation/**",
+ "restrictions": [
+ "**/test/automation/**",
+ "*" // node modules
+ ]
+ },
+ {
+ "target": "**/test/integration/**",
+ "restrictions": [
+ "**/test/integration/**",
+ "*" // node modules
+ ]
+ },
+ {
+ "target": "**/api/**.test.ts",
+ "restrictions": [
+ "**/vs/**",
+ "assert",
+ "sinon",
+ "crypto",
+ "vscode"
+ ]
+ },
+ {
+ "target": "**/{node,electron-browser,electron-main}/**/*.test.ts",
+ "restrictions": [
+ "**/vs/**",
+ "*" // node modules
+ ]
+ },
+ {
+ "target": "**/{node,electron-browser,electron-main}/**/test/**",
+ "restrictions": [
+ "**/vs/**",
+ "*" // node modules
+ ]
+ },
+ {
+ "target": "**/test/{node,electron-browser,electron-main}/**",
+ "restrictions": [
+ "**/vs/**",
+ "*" // node modules
+ ]
+ },
+ {
+ "target": "**/**.test.ts",
+ "restrictions": [
+ "**/vs/**",
+ "assert",
+ "sinon",
+ "crypto",
+ "xterm*"
+ ]
+ },
+ {
+ "target": "**/test/**",
+ "restrictions": [
+ "**/vs/**",
+ "assert",
+ "sinon",
+ "crypto",
+ "xterm*"
+ ]
+ }
+ ]
+ },
+ "overrides": [
+ {
+ "files": [
+ "*.js"
+ ],
+ "rules": {
+ "jsdoc/no-types": "off"
+ }
+ },
+ {
+ "files": [
+ "**/vscode.d.ts",
+ "**/vscode.proposed.d.ts"
+ ],
+ "rules": {
+ "vscode-dts-create-func": "warn",
+ "vscode-dts-literal-or-types": "warn",
+ "vscode-dts-interface-naming": "warn",
+ "vscode-dts-event-naming": [
+ "warn",
+ {
+ "allowed": [
+ "onCancellationRequested",
+ "event"
+ ],
+ "verbs": [
+ "accept",
+ "change",
+ "close",
+ "collapse",
+ "create",
+ "delete",
+ "dispose",
+ "edit",
+ "end",
+ "expand",
+ "hide",
+ "open",
+ "override",
+ "receive",
+ "register",
+ "rename",
+ "save",
+ "send",
+ "start",
+ "terminate",
+ "trigger",
+ "unregister",
+ "write"
+ ]
+ }
+ ]
+ }
+ }
+ ]
}
diff --git a/.github/ISSUE_TEMPLATE/bug_report.md b/.github/ISSUE_TEMPLATE/bug_report.md
index addf36f4339e4..acb1cb3d9c61f 100644
--- a/.github/ISSUE_TEMPLATE/bug_report.md
+++ b/.github/ISSUE_TEMPLATE/bug_report.md
@@ -2,7 +2,8 @@
name: Bug report
about: Create a report to help us improve
---
-
+
+
diff --git a/.github/ISSUE_TEMPLATE/feature_request.md b/.github/ISSUE_TEMPLATE/feature_request.md
index a820760fc48f7..b9c6c83caa3d9 100644
--- a/.github/ISSUE_TEMPLATE/feature_request.md
+++ b/.github/ISSUE_TEMPLATE/feature_request.md
@@ -4,6 +4,8 @@ about: Suggest an idea for this project
---
+
+
diff --git a/.github/classifier.json b/.github/classifier.json
new file mode 100644
index 0000000000000..33c179d323763
--- /dev/null
+++ b/.github/classifier.json
@@ -0,0 +1,182 @@
+{
+ "$schema": "https://raw.githubusercontent.com/microsoft/vscode-github-triage-actions/master/classifier-deep/apply/apply-labels/deep-classifier-config.schema.json",
+ "vacation": ["joaomoreno"],
+ "assignees": {
+ "JacksonKearl": {"accuracy": 0.5}
+ },
+ "labels": {
+ "L10N": {"assign": []},
+ "VIM": {"assign": []},
+ "api": {"assign": ["jrieken"]},
+ "api-finalization": {"assign": []},
+ "api-proposal": {"assign": ["jrieken"]},
+ "authentication": {"assign": ["RMacfarlane"]},
+ "breadcrumbs": {"assign": ["jrieken"]},
+ "callhierarchy": {"assign": ["jrieken"]},
+ "code-lens": {"assign": ["jrieken"]},
+ "color-palette": {"assign": []},
+ "comments": {"assign": ["rebornix"]},
+ "config": {"assign": ["sandy081"]},
+ "context-keys": {"assign": []},
+ "css-less-scss": {"assign": ["aeschli"]},
+ "custom-editors": {"assign": ["mjbvz"]},
+ "debug": {"assign": ["weinand"]},
+ "debug-console": {"assign": ["weinand"]},
+ "dialogs": {"assign": ["sbatten"]},
+ "diff-editor": {"assign": []},
+ "dropdown": {"assign": []},
+ "editor": {"assign": ["rebornix"]},
+ "editor-autoclosing": {"assign": []},
+ "editor-autoindent": {"assign": ["rebornix"]},
+ "editor-bracket-matching": {"assign": []},
+ "editor-clipboard": {"assign": ["jrieken"]},
+ "editor-code-actions": {"assign": []},
+ "editor-color-picker": {"assign": ["rebornix"]},
+ "editor-columnselect": {"assign": ["alexdima"]},
+ "editor-commands": {"assign": ["jrieken"]},
+ "editor-comments": {"assign": []},
+ "editor-contrib": {"assign": []},
+ "editor-core": {"assign": []},
+ "editor-drag-and-drop": {"assign": ["rebornix"]},
+ "editor-error-widget": {"assign": ["sandy081"]},
+ "editor-find": {"assign": ["rebornix"]},
+ "editor-folding": {"assign": ["aeschli"]},
+ "editor-hover": {"assign": []},
+ "editor-indent-guides": {"assign": []},
+ "editor-input": {"assign": ["alexdima"]},
+ "editor-input-IME": {"assign": ["rebornix"]},
+ "editor-minimap": {"assign": []},
+ "editor-multicursor": {"assign": ["alexdima"]},
+ "editor-parameter-hints": {"assign": []},
+ "editor-render-whitespace": {"assign": []},
+ "editor-rendering": {"assign": ["alexdima"]},
+ "editor-scrollbar": {"assign": []},
+ "editor-symbols": {"assign": ["jrieken"]},
+ "editor-synced-region": {"assign": ["aeschli"]},
+ "editor-textbuffer": {"assign": ["rebornix"]},
+ "editor-theming": {"assign": []},
+ "editor-wordnav": {"assign": ["alexdima"]},
+ "editor-wrapping": {"assign": ["alexdima"]},
+ "emmet": {"assign": []},
+ "error-list": {"assign": ["sandy081"]},
+ "explorer-custom": {"assign": ["sandy081"]},
+ "extension-host": {"assign": []},
+ "extensions": {"assign": ["sandy081"]},
+ "extensions-development": {"assign": []},
+ "file-decorations": {"assign": ["jrieken"]},
+ "file-encoding": {"assign": ["bpasero"]},
+ "file-explorer": {"assign": ["isidorn"]},
+ "file-glob": {"assign": []},
+ "file-guess-encoding": {"assign": ["bpasero"]},
+ "file-io": {"assign": ["bpasero"]},
+ "file-watcher": {"assign": ["bpasero"]},
+ "font-rendering": {"assign": []},
+ "formatting": {"assign": []},
+ "git": {"assign": ["joaomoreno"]},
+ "gpu": {"assign": ["deepak1556"]},
+ "grammar": {"assign": ["mjbvz"]},
+ "grid-view": {"assign": ["joaomoreno"]},
+ "html": {"assign": ["aeschli"]},
+ "i18n": {"assign": []},
+ "icon-brand": {"assign": []},
+ "icons-product": {"assign": ["misolori"]},
+ "install-update": {"assign": []},
+ "integrated-terminal": {"assign": ["Tyriar"]},
+ "integrated-terminal-conpty": {"assign": ["Tyriar"]},
+ "integrated-terminal-links": {"assign": ["Tyriar"]},
+ "integration-test": {"assign": []},
+ "intellisense-config": {"assign": []},
+ "ipc": {"assign": ["joaomoreno"]},
+ "issue-bot": {"assign": ["chrmarti"]},
+ "issue-reporter": {"assign": ["RMacfarlane"]},
+ "javascript": {"assign": ["mjbvz"]},
+ "json": {"assign": ["aeschli"]},
+ "keybindings": {"assign": []},
+ "keybindings-editor": {"assign": ["sandy081"]},
+ "keyboard-layout": {"assign": ["alexdima"]},
+ "languages-basic": {"assign": ["aeschli"]},
+ "languages-diagnostics": {"assign": ["jrieken"]},
+ "layout": {"assign": ["sbatten"]},
+ "lcd-text-rendering": {"assign": []},
+ "list": {"assign": ["joaomoreno"]},
+ "log": {"assign": []},
+ "markdown": {"assign": ["mjbvz"]},
+ "marketplace": {"assign": []},
+ "menus": {"assign": ["sbatten"]},
+ "merge-conflict": {"assign": ["chrmarti"]},
+ "notebook": {"assign": ["rebornix"]},
+ "outline": {"assign": ["jrieken"]},
+ "output": {"assign": []},
+ "perf": {"assign": []},
+ "perf-bloat": {"assign": []},
+ "perf-startup": {"assign": []},
+ "php": {"assign": ["roblourens"]},
+ "portable-mode": {"assign": ["joaomoreno"]},
+ "proxy": {"assign": []},
+ "quick-pick": {"assign": ["chrmarti"]},
+ "references-viewlet": {"assign": ["jrieken"]},
+ "release-notes": {"assign": []},
+ "remote": {"assign": []},
+ "remote-explorer": {"assign": ["alexr00"]},
+ "rename": {"assign": ["jrieken"]},
+ "scm": {"assign": ["joaomoreno"]},
+ "screencast-mode": {"assign": ["lszomoru"]},
+ "search": {"assign": ["roblourens"]},
+ "search-editor": {"assign": ["JacksonKearl"]},
+ "search-replace": {"assign": ["sandy081"]},
+ "semantic-tokens": {"assign": ["aeschli"]},
+ "settings-editor": {"assign": ["roblourens"]},
+ "settings-sync": {"assign": ["sandy081"]},
+ "simple-file-dialog": {"assign": ["alexr00"]},
+ "smart-select": {"assign": ["jrieken"]},
+ "smoke-test": {"assign": []},
+ "snap": {"assign": ["joaomoreno"]},
+ "snippets": {"assign": ["jrieken"]},
+ "splitview": {"assign": ["joaomoreno"]},
+ "suggest": {"assign": ["jrieken"]},
+ "tasks": {"assign": ["alexr00"], "accuracy": 0.85},
+ "telemetry": {"assign": []},
+ "themes": {"assign": ["aeschli"]},
+ "timeline": {"assign": ["eamodio"]},
+ "timeline-git": {"assign": ["eamodio"]},
+ "titlebar": {"assign": ["sbatten"]},
+ "tokenization": {"assign": []},
+ "tree": {"assign": ["joaomoreno"]},
+ "typescript": {"assign": ["mjbvz"]},
+ "undo-redo": {"assign": []},
+ "unit-test": {"assign": []},
+ "uri": {"assign": ["jrieken"]},
+ "ux": {"assign": ["misolori"]},
+ "variable-resolving": {"assign": []},
+ "vscode-build": {"assign": []},
+ "web": {"assign": ["bpasero"]},
+ "webview": {"assign": ["mjbvz"]},
+ "workbench-cli": {"assign": []},
+ "workbench-diagnostics": {"assign": ["RMacfarlane"]},
+ "workbench-dnd": {"assign": ["bpasero"]},
+ "workbench-editor-grid": {"assign": ["sbatten"]},
+ "workbench-editors": {"assign": ["bpasero"]},
+ "workbench-electron": {"assign": ["deepak1556"]},
+ "workbench-feedback": {"assign": ["bpasero"]},
+ "workbench-history": {"assign": ["bpasero"]},
+ "workbench-hot-exit": {"assign": ["Tyriar"]},
+ "workbench-launch": {"assign": []},
+ "workbench-link": {"assign": []},
+ "workbench-multiroot": {"assign": ["bpasero"]},
+ "workbench-notifications": {"assign": ["bpasero"]},
+ "workbench-os-integration": {"assign": []},
+ "workbench-rapid-render": {"assign": ["jrieken"]},
+ "workbench-run-as-admin": {"assign": []},
+ "workbench-state": {"assign": ["bpasero"]},
+ "workbench-status": {"assign": ["bpasero"]},
+ "workbench-tabs": {"assign": ["bpasero"]},
+ "workbench-touchbar": {"assign": ["bpasero"]},
+ "workbench-views": {"assign": ["sbatten"]},
+ "workbench-welcome": {"assign": ["chrmarti"]},
+ "workbench-window": {"assign": ["bpasero"]},
+ "workbench-zen": {"assign": ["isidorn"]},
+ "workspace-edit": {"assign": ["jrieken"]},
+ "workspace-symbols": {"assign": []},
+ "zoom": {"assign": ["alexdima"] }
+ }
+}
diff --git a/.github/classifier.yml b/.github/classifier.yml
deleted file mode 100644
index 92da2b03f5fb1..0000000000000
--- a/.github/classifier.yml
+++ /dev/null
@@ -1,309 +0,0 @@
-{
- perform: true,
- alwaysRequireAssignee: false,
- labelsRequiringAssignee: [],
- autoAssignees: {
- L10N: [],
- VIM: [],
- api: {
- assignees: [ jrieken ],
- assignLabel: false
- },
- cli: [],
- color-palette: [],
- config: [],
- css-less-scss: [],
- debug-console: [],
- debug: {
- assignees: [ weinand ],
- assignLabel: false
- },
- diff-editor: : {
- assignees: [],
- assignLabel: false
- },
- dropdown: [],
- editor: : {
- assignees: [],
- assignLabel: false
- },
- editor-1000-limit: : {
- assignees: [],
- assignLabel: false
- },
- editor-autoclosing: : {
- assignees: [],
- assignLabel: false
- },
- editor-autoindent: : {
- assignees: [],
- assignLabel: false
- },
- editor-brackets: : {
- assignees: [],
- assignLabel: false
- },
- editor-clipboard: : {
- assignees: [],
- assignLabel: false
- },
- editor-code-actions: : {
- assignees: [],
- assignLabel: false
- },
- editor-code-lens: : {
- assignees: [],
- assignLabel: false
- },
- editor-color-picker: : {
- assignees: [],
- assignLabel: false
- },
- editor-colors: : {
- assignees: [],
- assignLabel: false
- },
- editor-columnselect: : {
- assignees: [],
- assignLabel: false
- },
- editor-commands: : {
- assignees: [],
- assignLabel: false
- },
- editor-contrib: : {
- assignees: [],
- assignLabel: false
- },
- editor-drag-and-drop: : {
- assignees: [],
- assignLabel: false
- },
- editor-find: : {
- assignees: [],
- assignLabel: false
- },
- editor-folding: : {
- assignees: [],
- assignLabel: false
- },
- editor-hover: : {
- assignees: [],
- assignLabel: false
- },
- editor-ime: : {
- assignees: [],
- assignLabel: false
- },
- editor-input: : {
- assignees: [],
- assignLabel: false
- },
- editor-ligatures: : {
- assignees: [],
- assignLabel: false
- },
- editor-links: : {
- assignees: [],
- assignLabel: false
- },
- editor-minimap: : {
- assignees: [],
- assignLabel: false
- },
- editor-multicursor: : {
- assignees: [],
- assignLabel: false
- },
- editor-parameter-hints: : {
- assignees: [],
- assignLabel: false
- },
- editor-rendering: : {
- assignees: [],
- assignLabel: false
- },
- editor-smooth: : {
- assignees: [],
- assignLabel: false
- },
- editor-symbols: : {
- assignees: [],
- assignLabel: false
- },
- editor-textbuffer: : {
- assignees: [],
- assignLabel: false
- },
- editor-wrapping: : {
- assignees: [],
- assignLabel: false
- },
- emmet: [ octref ],
- error-list: [],
- explorer-custom: [],
- extension-host: [],
- extensions: [],
- extensions-development: [ octref ],
- file-decorations: [],
- file-encoding: {
- assignees: [],
- assignLabel: false
- },
- file-explorer: {
- assignees: [ isidorn ],
- assignLabel: false
- },
- file-glob: [],
- file-io: {
- assignees: [],
- assignLabel: false
- },
- file-watcher: {
- assignees: [],
- assignLabel: false
- },
- formatting: [],
- git: [],
- grammar: [],
- hot-exit: [],
- html: [],
- install-update: [],
- integrated-terminal: [],
- integration-test: [],
- intellisense-config: [],
- issue-reporter: [ RMacfarlane ],
- javascript: [ mjbvz ],
- json: [],
- keyboard-layout: : {
- assignees: [],
- assignLabel: false
- },
- keybindings: : {
- assignees: [],
- assignLabel: false
- },
- keybindings-editor: [],
- lang-diagnostics: [],
- languages basic: [],
- list: [],
- log: [],
- markdown: [ mjbvz ],
- marketplace: [],
- menus: [],
- merge-conflict: [ chrmarti ],
- multi-root: {
- assignees: [],
- assignLabel: false
- },
- os-integration: [],
- outline: [],
- output: [],
- perf-profile: [],
- perf-bloat: [],
- perf-startup: [],
- php: [ roblourens ],
- proxy: [],
- quick-pick: [ chrmarti ],
- release-notes: [],
- remote: {
- assignees: [ jrieken ],
- assignLabel: false
- },
- rename: [],
- run-as-admin: [],
- samples: [],
- scm: [],
- search: [ roblourens ],
- search-replace: [],
- settings-editor: [],
- shared-process: [],
- smart-select: [],
- smoke-test: [],
- snippets: {
- assignees: [ jrieken ],
- assignLabel: false
- },
- suggest: [],
- tasks: [ alexr00 ],
- telemetry: [],
- themes: [],
- tokenization: [],
- tree: [],
- typescript: [ mjbvz ],
- unit-test: [],
- uri: [],
- ux: [],
- vscode-build: [],
- webview: [],
- workbench: {
- assignees: [],
- assignLabel: false
- },
- workbench-diagnostics: {
- assignees: [],
- assignLabel: false
- },
- workbench-dnd: {
- assignees: [],
- assignLabel: false
- },
- workbench-editors: {
- assignees: [],
- assignLabel: false
- },
- workbench-electron: {
- assignees: [],
- assignLabel: false
- },
- workbench-feedback: {
- assignees: [],
- assignLabel: false
- },
- workbench-grid: {
- assignees: [],
- assignLabel: false
- },
- workbench-history: {
- assignees: [],
- assignLabel: false
- },
- workbench-layout: {
- assignees: [],
- assignLabel: false
- },
- workbench-menu: {
- assignees: [],
- assignLabel: false
- },
- workbench-notifications: {
- assignees: [],
- assignLabel: false
- },
- workbench-state: {
- assignees: [],
- assignLabel: false
- },
- workbench-status: {
- assignees: [],
- assignLabel: false
- },
- workbench-tabs: {
- assignees: [],
- assignLabel: false
- },
- workbench-title: {
- assignees: [],
- assignLabel: false
- },
- workbench-touchbar: {
- assignees: [],
- assignLabel: false
- },
- workbench-views: {
- assignees: [],
- assignLabel: false
- },
- workbench-welcome: [ chrmarti ]
- }
-}
diff --git a/.github/commands.json b/.github/commands.json
new file mode 100644
index 0000000000000..1b2bc516842d4
--- /dev/null
+++ b/.github/commands.json
@@ -0,0 +1,381 @@
+[
+ {
+ "type": "comment",
+ "name": "question",
+ "allowUsers": [
+ "cleidigh",
+ "usernamehw",
+ "gjsjohnmurray",
+ "IllusionMH"
+ ],
+ "action": "updateLabels",
+ "addLabel": "*question"
+ },
+ {
+ "type": "label",
+ "name": "*question",
+ "action": "close",
+ "comment": "Please ask your question on [StackOverflow](https://aka.ms/vscodestackoverflow). We have a great community over [there](https://aka.ms/vscodestackoverflow). They have already answered thousands of questions and are happy to answer yours as well. See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines.\n\nHappy Coding!"
+ },
+ {
+ "type": "label",
+ "name": "*dev-question",
+ "action": "close",
+ "comment": "We have a great developer community [over on slack](https://aka.ms/vscode-dev-community) where extension authors help each other. This is a great place for you to ask questions and find support.\n\nHappy Coding!"
+ },
+ {
+ "type": "label",
+ "name": "*extension-candidate",
+ "action": "close",
+ "comment": "We try to keep VS Code lean and we think the functionality you're asking for is great for a VS Code extension. Maybe you can already find one that suits you in the [VS Code Marketplace](https://aka.ms/vscodemarketplace). Just in case, in a few simple steps you can get started [writing your own extension](https://aka.ms/vscodewritingextensions). See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines.\n\nHappy Coding!"
+ },
+ {
+ "type": "label",
+ "name": "*not-reproducible",
+ "action": "close",
+ "comment": "We closed this issue because we are unable to reproduce the problem with the steps you describe. Chances are we've already fixed your problem in a recent version of VS Code. If not, please ask us to reopen the issue and provide us with more detail. Our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines might help you with that.\n\nHappy Coding!"
+ },
+ {
+ "type": "label",
+ "name": "*out-of-scope",
+ "action": "close",
+ "comment": "We closed this issue because we don't plan to address it in the foreseeable future. You can find more detailed information about our decision-making process [here](https://aka.ms/vscode-out-of-scope). If you disagree and feel that this issue is crucial: We are happy to listen and to reconsider.\n\nIf you wonder what we are up to, please see our [roadmap](https://aka.ms/vscoderoadmap) and [issue reporting](https://aka.ms/vscodeissuereporting) guidelines.\n\nThanks for your understanding and happy coding!"
+ },
+ {
+ "type": "comment",
+ "name": "causedByExtension",
+ "allowUsers": [
+ "cleidigh",
+ "usernamehw",
+ "gjsjohnmurray",
+ "IllusionMH"
+ ],
+ "action": "updateLabels",
+ "addLabel": "*caused-by-extension"
+ },
+ {
+ "type": "label",
+ "name": "*caused-by-extension",
+ "action": "close",
+ "comment": "This issue is caused by an extension, please file it with the repository (or contact) the extension has linked in its overview in VS Code or the [marketplace](https://aka.ms/vscodemarketplace) for VS Code. See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines.\n\nHappy Coding!"
+ },
+ {
+ "type": "label",
+ "name": "*as-designed",
+ "action": "close",
+ "comment": "The described behavior is how it is expected to work. If you disagree, please explain what is expected and what is not in more detail. See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines.\n\nHappy Coding!"
+ },
+ {
+ "type": "comment",
+ "name": "duplicate",
+ "allowUsers": [
+ "cleidigh",
+ "usernamehw",
+ "gjsjohnmurray",
+ "IllusionMH"
+ ],
+ "action": "updateLabels",
+ "addLabel": "*duplicate"
+ },
+ {
+ "type": "label",
+ "name": "*duplicate",
+ "action": "close",
+ "comment": "Thanks for creating this issue! We figured it's covering the same as another one we already have. Thus, we closed this one as a duplicate. You can search for existing issues [here](https://aka.ms/vscodeissuesearch). See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines.\n\nHappy Coding!"
+ },
+ {
+ "type": "comment",
+ "name": "verified",
+ "allowUsers": [
+ "@author"
+ ],
+ "action": "updateLabels",
+ "addLabel": "z-author-verified",
+ "removeLabel": "author-verification-requested",
+ "requireLabel": "author-verification-requested",
+ "disallowLabel": "awaiting-insiders-release"
+ },
+ {
+ "type": "comment",
+ "name": "confirm",
+ "allowUsers": [
+ "cleidigh",
+ "usernamehw",
+ "gjsjohnmurray",
+ "IllusionMH"
+ ],
+ "action": "updateLabels",
+ "addLabel": "confirmed",
+ "removeLabel": "confirmation-pending"
+ },
+ {
+ "type": "comment",
+ "name": "confirmationPending",
+ "allowUsers": [
+ "cleidigh",
+ "usernamehw",
+ "gjsjohnmurray",
+ "IllusionMH"
+ ],
+ "action": "updateLabels",
+ "addLabel": "confirmation-pending",
+ "removeLabel": "confirmed"
+ },
+ {
+ "type": "comment",
+ "name": "needsMoreInfo",
+ "allowUsers": [
+ "cleidigh",
+ "usernamehw",
+ "gjsjohnmurray",
+ "IllusionMH"
+ ],
+ "action": "updateLabels",
+ "addLabel": "~needs more info"
+ },
+ {
+ "type": "comment",
+ "name": "closedWith",
+ "allowUsers": [
+ "cleidigh",
+ "usernamehw",
+ "gjsjohnmurray",
+ "IllusionMH"
+ ],
+ "action": "close",
+ "addLabel": "unreleased"
+ },
+ {
+ "type": "label",
+ "name": "~needs more info",
+ "action": "updateLabels",
+ "addLabel": "needs more info",
+ "removeLabel": "~needs more info",
+ "comment": "Thanks for creating this issue! We figured it's missing some basic information or in some other way doesn't follow our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines. Please take the time to review these and update the issue.\n\nHappy Coding!"
+ },
+ {
+ "type": "label",
+ "name": "~needs version info",
+ "action": "updateLabels",
+ "addLabel": "needs more info",
+ "removeLabel": "~needs version info",
+ "comment": "Thanks for creating this issue! We figured it's missing some basic information, such as a version number, or in some other way doesn't follow our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines. Please take the time to review these and update the issue.\n\nHappy Coding!"
+ },
+ {
+ "type": "comment",
+ "name": "a11ymas",
+ "allowUsers": [
+ "AccessibilityTestingTeam-TCS",
+ "dixitsonali95",
+ "Mohini78",
+ "ChitrarupaSharma",
+ "mspatil110",
+ "umasarath52",
+ "v-umnaik"
+ ],
+ "action": "updateLabels",
+ "addLabel": "a11ymas"
+ },
+ {
+ "type": "label",
+ "name": "*off-topic",
+ "action": "close",
+ "comment": "Thanks for creating this issue. We think this issue is unactionable or unrelated to the goals of this project. Please follow our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines.\n\nHappy Coding!"
+ },
+ {
+ "type": "comment",
+ "name": "extPython",
+ "allowUsers": [
+ "cleidigh",
+ "usernamehw",
+ "gjsjohnmurray",
+ "IllusionMH"
+ ],
+ "action": "close",
+ "addLabel": "*caused-by-extension",
+ "comment": "It looks like this is caused by the Python extension. Please file it with the repository [here](https://github.com/Microsoft/vscode-python). Make sure to check their issue reporting template and provide them relevant information such as the extension version you're using. See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines for more information.\n\nHappy Coding!"
+ },
+ {
+ "type": "comment",
+ "name": "extC",
+ "allowUsers": [
+ "cleidigh",
+ "usernamehw",
+ "gjsjohnmurray",
+ "IllusionMH"
+ ],
+ "action": "close",
+ "addLabel": "*caused-by-extension",
+ "comment": "It looks like this is caused by the C extension. Please file it with the repository [here](https://github.com/Microsoft/vscode-cpptools). Make sure to check their issue reporting template and provide them relevant information such as the extension version you're using. See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines for more information.\n\nHappy Coding!"
+ },
+ {
+ "type": "comment",
+ "name": "extC++",
+ "allowUsers": [
+ "cleidigh",
+ "usernamehw",
+ "gjsjohnmurray",
+ "IllusionMH"
+ ],
+ "action": "close",
+ "addLabel": "*caused-by-extension",
+ "comment": "It looks like this is caused by the C++ extension. Please file it with the repository [here](https://github.com/Microsoft/vscode-cpptools). Make sure to check their issue reporting template and provide them relevant information such as the extension version you're using. See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines for more information.\n\nHappy Coding!"
+ },
+ {
+ "type": "comment",
+ "name": "extCpp",
+ "allowUsers": [
+ "cleidigh",
+ "usernamehw",
+ "gjsjohnmurray",
+ "IllusionMH"
+ ],
+ "action": "close",
+ "addLabel": "*caused-by-extension",
+ "comment": "It looks like this is caused by the C++ extension. Please file it with the repository [here](https://github.com/Microsoft/vscode-cpptools). Make sure to check their issue reporting template and provide them relevant information such as the extension version you're using. See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines for more information.\n\nHappy Coding!"
+ },
+ {
+ "type": "comment",
+ "name": "extTS",
+ "allowUsers": [
+ "cleidigh",
+ "usernamehw",
+ "gjsjohnmurray",
+ "IllusionMH"
+ ],
+ "action": "close",
+ "addLabel": "*caused-by-extension",
+ "comment": "It looks like this is caused by the TypeScript language service. Please file it with the repository [here](https://github.com/microsoft/TypeScript/). Make sure to check their [contributing guidelines](https://github.com/microsoft/TypeScript/blob/master/CONTRIBUTING.md) and provide relevant information such as the extension version you're using. See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines for more information.\n\nHappy Coding!"
+ },
+ {
+ "type": "comment",
+ "name": "extJS",
+ "allowUsers": [
+ "cleidigh",
+ "usernamehw",
+ "gjsjohnmurray",
+ "IllusionMH"
+ ],
+ "action": "close",
+ "addLabel": "*caused-by-extension",
+ "comment": "It looks like this is caused by the TypeScript/JavaScript language service. Please file it with the repository [here](https://github.com/microsoft/TypeScript/). Make sure to check their [contributing guidelines](https://github.com/microsoft/TypeScript/blob/master/CONTRIBUTING.md) and provide relevant information such as the extension version you're using. See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines for more information.\n\nHappy Coding!"
+ },
+ {
+ "type": "comment",
+ "name": "extC#",
+ "allowUsers": [
+ "cleidigh",
+ "usernamehw",
+ "gjsjohnmurray",
+ "IllusionMH"
+ ],
+ "action": "close",
+ "addLabel": "*caused-by-extension",
+ "comment": "It looks like this is caused by the C# extension. Please file it with the repository [here](https://github.com/OmniSharp/omnisharp-vscode.git). Make sure to check their issue reporting template and provide them relevant information such as the extension version you're using. See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines for more information.\n\nHappy Coding!"
+ },
+ {
+ "type": "comment",
+ "name": "extGo",
+ "allowUsers": [
+ "cleidigh",
+ "usernamehw",
+ "gjsjohnmurray",
+ "IllusionMH"
+ ],
+ "action": "close",
+ "addLabel": "*caused-by-extension",
+ "comment": "It looks like this is caused by the Go extension. Please file it with the repository [here](https://github.com/golang/vscode-go). Make sure to check their issue reporting template and provide them relevant information such as the extension version you're using. See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines for more information.\n\nHappy Coding!"
+ },
+ {
+ "type": "comment",
+ "name": "extPowershell",
+ "allowUsers": [
+ "cleidigh",
+ "usernamehw",
+ "gjsjohnmurray",
+ "IllusionMH"
+ ],
+ "action": "close",
+ "addLabel": "*caused-by-extension",
+ "comment": "It looks like this is caused by the PowerShell extension. Please file it with the repository [here](https://github.com/PowerShell/vscode-powershell). Make sure to check their issue reporting template and provide them relevant information such as the extension version you're using. See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines for more information.\n\nHappy Coding!"
+ },
+ {
+ "type": "comment",
+ "name": "extLiveShare",
+ "allowUsers": [
+ "cleidigh",
+ "usernamehw",
+ "gjsjohnmurray",
+ "IllusionMH"
+ ],
+ "action": "close",
+ "addLabel": "*caused-by-extension",
+ "comment": "It looks like this is caused by the LiveShare extension. Please file it with the repository [here](https://github.com/MicrosoftDocs/live-share). Make sure to check their [contributing guidelines](https://github.com/MicrosoftDocs/live-share/blob/master/CONTRIBUTING.md) and provide relevant information such as the extension version you're using. See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines for more information.\n\nHappy Coding!"
+ },
+ {
+ "type": "comment",
+ "name": "extDocker",
+ "allowUsers": [
+ "cleidigh",
+ "usernamehw",
+ "gjsjohnmurray",
+ "IllusionMH"
+ ],
+ "action": "close",
+ "addLabel": "*caused-by-extension",
+ "comment": "It looks like this is caused by the Docker extension. Please file it with the repository [here](https://github.com/microsoft/vscode-docker). Make sure to check their issue reporting template and provide them relevant information such as the extension version you're using. See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines for more information.\n\nHappy Coding!"
+ },
+ {
+ "type": "comment",
+ "name": "extJava",
+ "allowUsers": [
+ "cleidigh",
+ "usernamehw",
+ "gjsjohnmurray",
+ "IllusionMH"
+ ],
+ "action": "close",
+ "addLabel": "*caused-by-extension",
+ "comment": "It looks like this is caused by the Java extension. Please file it with the repository [here](https://github.com/redhat-developer/vscode-java). Make sure to check their [troubleshooting instructions](https://github.com/redhat-developer/vscode-java/wiki/Troubleshooting) and provide relevant information such as the extension version you're using. See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines for more information.\n\nHappy Coding!"
+ },
+ {
+ "type": "comment",
+ "name": "extJavaDebug",
+ "allowUsers": [
+ "cleidigh",
+ "usernamehw",
+ "gjsjohnmurray",
+ "IllusionMH"
+ ],
+ "action": "close",
+ "addLabel": "*caused-by-extension",
+ "comment": "It looks like this is caused by the Java Debugger extension. Please file it with the repository [here](https://github.com/Microsoft/vscode-java-debug). Make sure to check their issue reporting template and provide them relevant information such as the extension version you're using. See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines for more information.\n\nHappy Coding!"
+ },
+ {
+ "type": "comment",
+ "name": "gifPlease",
+ "allowUsers": [
+ "cleidigh",
+ "usernamehw",
+ "gjsjohnmurray",
+ "IllusionMH"
+ ],
+ "action": "comment",
+ "comment": "Thanks for reporting this issue! Unfortunately, it's hard for us to understand what issue you're seeing. Please help us out by providing a screen recording showing exactly what isn't working as expected. While we can work with most standard formats, `.gif` files are preferred as they are displayed inline on GitHub. You may find https://gifcap.dev helpful as a browser-based gif recording tool.\n\nIf the issue depends on keyboard input, you can help us by enabling screencast mode for the recording (`Developer: Toggle Screencast Mode` in the command palette).\n\nHappy coding!"
+ },
+ {
+ "type": "comment",
+ "name": "label",
+ "allowUsers": []
+ },
+ {
+ "type": "comment",
+ "name": "assign",
+ "allowUsers": [
+ "cleidigh",
+ "usernamehw",
+ "gjsjohnmurray",
+ "IllusionMH"
+ ]
+ }
+]
diff --git a/.github/commands.yml b/.github/commands.yml
index 0e78ac749fafa..64fdf683bfe99 100644
--- a/.github/commands.yml
+++ b/.github/commands.yml
@@ -1,127 +1,12 @@
{
perform: true,
commands: [
- {
- type: 'comment',
- name: 'question',
- allowUsers: ['cleidigh', 'usernamehw', 'gjsjohnmurray', 'IllusionMH'],
- action: 'updateLabels',
- addLabel: '*question'
- },
- {
- type: 'label',
- name: '*question',
- allowTriggerByBot: true,
- action: 'close',
- comment: "Please ask your question on [StackOverflow](https://aka.ms/vscodestackoverflow). We have a great community over [there](https://aka.ms/vscodestackoverflow). They have already answered thousands of questions and are happy to answer yours as well. See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines.\n\nHappy Coding!"
- },
- {
- type: 'label',
- name: '*dev-question',
- allowTriggerByBot: true,
- action: 'close',
- comment: "We have a great developer community [over on slack](https://aka.ms/vscode-dev-community) where extension authors help each other. This is a great place for you to ask questions and find support.\n\nHappy Coding!"
- },
- {
- type: 'label',
- name: '*extension-candidate',
- allowTriggerByBot: true,
- action: 'close',
- comment: "We try to keep VS Code lean and we think the functionality you're asking for is great for a VS Code extension. Maybe you can already find one that suits you in the [VS Code Marketplace](https://aka.ms/vscodemarketplace). Just in case, in a few simple steps you can get started [writing your own extension](https://aka.ms/vscodewritingextensions). See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines.\n\nHappy Coding!"
- },
- {
- type: 'label',
- name: '*not-reproducible',
- allowTriggerByBot: true,
- action: 'close',
- comment: "We closed this issue because we are unable to reproduce the problem with the steps you describe. Chances are we've already fixed your problem in a recent version of VS Code. If not, please ask us to reopen the issue and provide us with more detail. Our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines might help you with that.\n\nHappy Coding!"
- },
- {
- type: 'label',
- name: '*out-of-scope',
- allowTriggerByBot: true,
- action: 'close',
- comment: "We closed this issue because we don't plan to address it in the foreseeable future. You can find more detailed information about our decision-making process [here](https://aka.ms/vscode-out-of-scope). If you disagree and feel that this issue is crucial: We are happy to listen and to reconsider.\n\nIf you wonder what we are up to, please see our [roadmap](https://aka.ms/vscoderoadmap) and [issue reporting](https://aka.ms/vscodeissuereporting) guidelines.\n\nThanks for your understanding and happy coding!"
- },
- {
- type: 'comment',
- name: 'causedByExtension',
- allowUsers: ['cleidigh', 'usernamehw', 'gjsjohnmurray', 'IllusionMH'],
- action: 'updateLabels',
- addLabel: '*caused-by-extension'
- },
- {
- type: 'label',
- name: '*caused-by-extension',
- allowTriggerByBot: true,
- action: 'close',
- comment: "This issue is caused by an extension, please file it with the repository (or contact) the extension has linked in its overview in VS Code or the [marketplace](https://aka.ms/vscodemarketplace) for VS Code. See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines.\n\nHappy Coding!"
- },
- {
- type: 'label',
- name: '*as-designed',
- allowTriggerByBot: true,
- action: 'close',
- comment: "The described behavior is how it is expected to work. If you disagree, please explain what is expected and what is not in more detail. See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines.\n\nHappy Coding!"
- },
- {
- type: 'label',
- name: '*english-please',
- allowTriggerByBot: true,
- action: 'close',
- comment: "This issue is being closed because its description is not in English, that makes it hard for us to work on it. Please open a new issue with an English description. You might find [Bing Translator](https://www.bing.com/translator) useful."
- },
- {
- type: 'comment',
- name: 'duplicate',
- allowUsers: ['cleidigh', 'usernamehw', 'gjsjohnmurray', 'IllusionMH'],
- action: 'updateLabels',
- addLabel: '*duplicate'
- },
- {
- type: 'label',
- name: '*duplicate',
- allowTriggerByBot: true,
- action: 'close',
- comment: "Thanks for creating this issue! We figured it's covering the same as another one we already have. Thus, we closed this one as a duplicate. You can search for existing issues [here](https://aka.ms/vscodeissuesearch). See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines.\n\nHappy Coding!"
- },
- {
- type: 'comment',
- name: 'confirm',
- allowUsers: ['cleidigh', 'usernamehw', 'gjsjohnmurray', 'IllusionMH'],
- action: 'updateLabels',
- addLabel: 'confirmed',
- removeLabel: 'confirmation-pending'
- },
- {
- type: 'comment',
- name: 'confirmationPending',
- allowUsers: ['cleidigh', 'usernamehw'],
- action: 'updateLabels',
- addLabel: 'confirmation-pending',
- removeLabel: 'confirmed'
- },
{
type: 'comment',
name: 'findDuplicates',
allowUsers: ['cleidigh', 'usernamehw', 'gjsjohnmurray', 'IllusionMH'],
action: 'comment',
comment: "Potential duplicates:\n${potentialDuplicates}"
- },
- {
- type: 'comment',
- name: 'needsMoreInfo',
- allowUsers: ['cleidigh', 'usernamehw', 'gjsjohnmurray', 'IllusionMH'],
- action: 'updateLabels',
- addLabel: 'needs more info',
- comment: "Thanks for creating this issue! We figured it's missing some basic information or in some other way doesn't follow our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines. Please take the time to review these and update the issue.\n\nHappy Coding!"
- },
- {
- type: 'comment',
- name: 'a11ymas',
- allowUsers: ['AccessibilityTestingTeam-TCS', 'dixitsonali95', 'Mohini78', 'ChitrarupaSharma', 'mspatil110', 'umasarath52', 'v-umnaik'],
- action: 'updateLabels',
- addLabel: 'a11ymas'
- },
+ }
]
}
diff --git a/.github/copycat.yml b/.github/copycat.yml
deleted file mode 100644
index eccccc16b007d..0000000000000
--- a/.github/copycat.yml
+++ /dev/null
@@ -1,5 +0,0 @@
-{
- perform: true,
- target_owner: 'chrmarti',
- target_repo: 'testissues'
-}
\ No newline at end of file
diff --git a/.github/locker.yml b/.github/locker.yml
deleted file mode 100644
index 6d8feccae14c6..0000000000000
--- a/.github/locker.yml
+++ /dev/null
@@ -1,6 +0,0 @@
-{
- daysAfterClose: 45,
- daysSinceLastUpdate: 3,
- ignoredLabels: ['*out-of-scope'],
- perform: true
-}
diff --git a/.github/needs_more_info.yml b/.github/needs_more_info.yml
deleted file mode 100644
index e5b2cddd8800c..0000000000000
--- a/.github/needs_more_info.yml
+++ /dev/null
@@ -1,6 +0,0 @@
-{
- daysUntilClose: 7,
- needsMoreInfoLabel: 'needs more info',
- perform: true,
- closeComment: "This issue has been closed automatically because it needs more information and has not had recent activity. See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines.\n\nHappy Coding!"
-}
diff --git a/.github/new_release.yml b/.github/new_release.yml
deleted file mode 100644
index 7482b60b108ea..0000000000000
--- a/.github/new_release.yml
+++ /dev/null
@@ -1,6 +0,0 @@
-{
- newReleaseLabel: 'new release',
- newReleaseColor: '006b75',
- daysAfterRelease: 5,
- perform: true
-}
diff --git a/.github/subscribers.json b/.github/subscribers.json
new file mode 100644
index 0000000000000..89dee80d4a9f5
--- /dev/null
+++ b/.github/subscribers.json
@@ -0,0 +1,7 @@
+{
+ "label-to-subscribe-to": [
+ "list of usernames to subscribe",
+ "such as:",
+ "JacksonKearl"
+ ]
+}
diff --git a/.github/workflows/author-verified.yml b/.github/workflows/author-verified.yml
new file mode 100644
index 0000000000000..03cd7cedbef3f
--- /dev/null
+++ b/.github/workflows/author-verified.yml
@@ -0,0 +1,39 @@
+name: Author Verified
+on:
+ repository_dispatch:
+ types: [trigger-author-verified]
+ schedule:
+ - cron: 20 14 * * * # 4:20pm Zurich
+ issues:
+ types: [closed]
+
+# also make changes in ./on-label.yml
+jobs:
+ main:
+ runs-on: ubuntu-latest
+ steps:
+ - name: Checkout Actions
+ if: github.event_name != 'issues' || contains(github.event.issue.labels.*.name, 'author-verification-requested')
+ uses: actions/checkout@v2
+ with:
+ repository: 'microsoft/vscode-github-triage-actions'
+ ref: v35
+ path: ./actions
+ - name: Install Actions
+ if: github.event_name != 'issues' || contains(github.event.issue.labels.*.name, 'author-verification-requested')
+ run: npm install --production --prefix ./actions
+ - name: Checkout Repo
+ if: github.event_name != 'issues' || contains(github.event.issue.labels.*.name, 'author-verification-requested')
+ uses: actions/checkout@v2
+ with:
+ path: ./repo
+ fetch-depth: 0
+ - name: Run Author Verified
+ if: github.event_name != 'issues' || contains(github.event.issue.labels.*.name, 'author-verification-requested')
+ uses: ./actions/author-verified
+ with:
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ token: ${{secrets.VSCODE_ISSUE_TRIAGE_BOT_PAT}}
+ requestVerificationComment: "This bug has been fixed in to the latest release of [VS Code Insiders](https://code.visualstudio.com/insiders/)!\n\n@${author}, you can help us out by commenting `/verified` if things are now working as expected.\n\nIf things still don't seem right, please ensure you're on version ${commit} of Insiders (today's or later - you can use `Help: About` in the command pallette to check), and leave a comment letting us know what isn't working as expected.\n\nHappy Coding!"
+ pendingReleaseLabel: awaiting-insiders-release
+ authorVerificationRequestedLabel: author-verification-requested
diff --git a/.github/workflows/ci.yml b/.github/workflows/ci.yml
index 55415410f7cd5..d509cce2a33e0 100644
--- a/.github/workflows/ci.yml
+++ b/.github/workflows/ci.yml
@@ -11,7 +11,113 @@ on:
- release/*
jobs:
- linux:
+ # linux:
+ # runs-on: ubuntu-latest
+ # env:
+ # CHILD_CONCURRENCY: "1"
+ # GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }}
+ # steps:
+ # - uses: actions/checkout@v1
+ # # TODO: rename azure-pipelines/linux/xvfb.init to github-actions
+ # - run: |
+ # sudo apt-get update
+ # sudo apt-get install -y libxkbfile-dev pkg-config libsecret-1-dev libxss1 dbus xvfb libgtk-3-0 libgbm1
+ # sudo cp build/azure-pipelines/linux/xvfb.init /etc/init.d/xvfb
+ # sudo chmod +x /etc/init.d/xvfb
+ # sudo update-rc.d xvfb defaults
+ # sudo service xvfb start
+ # name: Setup Build Environment
+ # - uses: actions/setup-node@v1
+ # with:
+ # node-version: 10
+ # # TODO: cache node modules
+ # - run: yarn --frozen-lockfile
+ # name: Install Dependencies
+ # - run: yarn electron x64
+ # name: Download Electron
+ # - run: yarn gulp hygiene
+ # name: Run Hygiene Checks
+ # - run: yarn monaco-compile-check
+ # name: Run Monaco Editor Checks
+ # - run: yarn valid-layers-check
+ # name: Run Valid Layers Checks
+ # - run: yarn compile
+ # name: Compile Sources
+ # - run: yarn download-builtin-extensions
+ # name: Download Built-in Extensions
+ # - run: DISPLAY=:10 ./scripts/test.sh --tfs "Unit Tests"
+ # name: Run Unit Tests (Electron)
+ # - run: DISPLAY=:10 yarn test-browser --browser chromium
+ # name: Run Unit Tests (Browser)
+ # - run: DISPLAY=:10 ./scripts/test-integration.sh --tfs "Integration Tests"
+ # name: Run Integration Tests (Electron)
+
+ # windows:
+ # runs-on: windows-2016
+ # env:
+ # CHILD_CONCURRENCY: "1"
+ # GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }}
+ # steps:
+ # - uses: actions/checkout@v1
+ # - uses: actions/setup-node@v1
+ # with:
+ # node-version: 10
+ # - uses: actions/setup-python@v1
+ # with:
+ # python-version: '2.x'
+ # - run: yarn --frozen-lockfile
+ # name: Install Dependencies
+ # - run: yarn electron
+ # name: Download Electron
+ # - run: yarn gulp hygiene
+ # name: Run Hygiene Checks
+ # - run: yarn monaco-compile-check
+ # name: Run Monaco Editor Checks
+ # - run: yarn valid-layers-check
+ # name: Run Valid Layers Checks
+ # - run: yarn compile
+ # name: Compile Sources
+ # - run: yarn download-builtin-extensions
+ # name: Download Built-in Extensions
+ # - run: .\scripts\test.bat --tfs "Unit Tests"
+ # name: Run Unit Tests (Electron)
+ # - run: yarn test-browser --browser chromium
+ # name: Run Unit Tests (Browser)
+ # - run: .\scripts\test-integration.bat --tfs "Integration Tests"
+ # name: Run Integration Tests (Electron)
+
+ # darwin:
+ # runs-on: macos-latest
+ # env:
+ # CHILD_CONCURRENCY: "1"
+ # GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }}
+ # steps:
+ # - uses: actions/checkout@v1
+ # - uses: actions/setup-node@v1
+ # with:
+ # node-version: 10
+ # - run: yarn --frozen-lockfile
+ # name: Install Dependencies
+ # - run: yarn electron x64
+ # name: Download Electron
+ # - run: yarn gulp hygiene
+ # name: Run Hygiene Checks
+ # - run: yarn monaco-compile-check
+ # name: Run Monaco Editor Checks
+ # - run: yarn valid-layers-check
+ # name: Run Valid Layers Checks
+ # - run: yarn compile
+ # name: Compile Sources
+ # - run: yarn download-builtin-extensions
+ # name: Download Built-in Extensions
+ # - run: ./scripts/test.sh --tfs "Unit Tests"
+ # name: Run Unit Tests (Electron)
+ # - run: yarn test-browser --browser chromium --browser webkit
+ # name: Run Unit Tests (Browser)
+ # - run: ./scripts/test-integration.sh --tfs "Integration Tests"
+ # name: Run Integration Tests (Electron)
+
+ monaco:
runs-on: ubuntu-latest
env:
CHILD_CONCURRENCY: "1"
@@ -21,92 +127,18 @@ jobs:
# TODO: rename azure-pipelines/linux/xvfb.init to github-actions
- run: |
sudo apt-get update
- sudo apt-get install -y libxkbfile-dev pkg-config libsecret-1-dev libxss1 dbus xvfb libgtk-3-0
+ sudo apt-get install -y libxkbfile-dev pkg-config libsecret-1-dev libxss1 dbus xvfb libgtk-3-0 libgbm1
sudo cp build/azure-pipelines/linux/xvfb.init /etc/init.d/xvfb
sudo chmod +x /etc/init.d/xvfb
sudo update-rc.d xvfb defaults
sudo service xvfb start
name: Setup Build Environment
- - uses: actions/setup-node@v1
- with:
- node-version: 10
- # TODO: cache node modules
- - run: yarn --frozen-lockfile
- name: Install Dependencies
- - run: yarn electron x64
- name: Download Electron
- - run: yarn gulp hygiene --skip-tslint
- name: Run Hygiene Checks
- - run: yarn gulp tslint
- name: Run TSLint Checks
- - run: yarn monaco-compile-check
- name: Run Monaco Editor Checks
- - run: yarn compile
- name: Compile Sources
- - run: yarn download-builtin-extensions
- name: Download Built-in Extensions
- - run: DISPLAY=:10 ./scripts/test.sh --tfs "Unit Tests"
- name: Run Unit Tests
- - run: DISPLAY=:10 ./scripts/test-integration.sh --tfs "Integration Tests"
- name: Run Integration Tests
-
- windows:
- runs-on: windows-2016
- env:
- CHILD_CONCURRENCY: "1"
- GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }}
- steps:
- - uses: actions/checkout@v1
- - uses: actions/setup-node@v1
- with:
- node-version: 10
- - uses: actions/setup-python@v1
- with:
- python-version: '2.x'
- - run: yarn --frozen-lockfile
- name: Install Dependencies
- - run: yarn electron
- name: Download Electron
- - run: yarn gulp hygiene --skip-tslint
- name: Run Hygiene Checks
- - run: yarn gulp tslint
- name: Run TSLint Checks
- - run: yarn monaco-compile-check
- name: Run Monaco Editor Checks
- - run: yarn compile
- name: Compile Sources
- - run: yarn download-builtin-extensions
- name: Download Built-in Extensions
- - run: .\scripts\test.bat --tfs "Unit Tests"
- name: Run Unit Tests
- - run: .\scripts\test-integration.bat --tfs "Integration Tests"
- name: Run Integration Tests
-
- darwin:
- runs-on: macos-latest
- env:
- CHILD_CONCURRENCY: "1"
- GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }}
- steps:
- - uses: actions/checkout@v1
- uses: actions/setup-node@v1
with:
node-version: 10
- run: yarn --frozen-lockfile
name: Install Dependencies
- - run: yarn electron x64
- name: Download Electron
- - run: yarn gulp hygiene --skip-tslint
- name: Run Hygiene Checks
- - run: yarn gulp tslint
- name: Run TSLint Checks
- run: yarn monaco-compile-check
name: Run Monaco Editor Checks
- - run: yarn compile
- name: Compile Sources
- - run: yarn download-builtin-extensions
- name: Download Built-in Extensions
- - run: ./scripts/test.sh --tfs "Unit Tests"
- name: Run Unit Tests
- - run: ./scripts/test-integration.sh --tfs "Integration Tests"
- name: Run Integration Tests
+ - run: yarn gulp editor-esm-bundle
+ name: Editor Distro & ESM Bundle
diff --git a/.github/workflows/codeql.yml b/.github/workflows/codeql.yml
new file mode 100644
index 0000000000000..017708844a471
--- /dev/null
+++ b/.github/workflows/codeql.yml
@@ -0,0 +1,49 @@
+name: "Code Scanning"
+
+on:
+ schedule:
+ - cron: '0 0 * * 2'
+
+jobs:
+ CodeQL-Build:
+
+ # CodeQL runs on ubuntu-latest and windows-latest
+ runs-on: ubuntu-latest
+
+ steps:
+ - name: Checkout repository
+ uses: actions/checkout@v2
+ with:
+ # We must fetch at least the immediate parents so that if this is
+ # a pull request then we can checkout the head.
+ fetch-depth: 2
+
+ # If this run was triggered by a pull request event, then checkout
+ # the head of the pull request instead of the merge commit.
+ - run: git checkout HEAD^2
+ if: ${{ github.event_name == 'pull_request' }}
+
+ # Initializes the CodeQL tools for scanning.
+ - name: Initialize CodeQL
+ uses: github/codeql-action/init@v1
+ with:
+ languages: javascript
+
+ # Autobuild attempts to build any compiled languages (C/C++, C#, or Java).
+ # If this step fails, then you should remove it and run the build manually (see below)
+ - name: Autobuild
+ uses: github/codeql-action/autobuild@v1
+
+ # ℹ️ Command-line programs to run using the OS shell.
+ # 📚 https://git.io/JvXDl
+
+ # ✏️ If the Autobuild fails above, remove it and uncomment the following three lines
+ # and modify them (or add more) to build your code if your project
+ # uses a compiled language
+
+ #- run: |
+ # make bootstrap
+ # make release
+
+ - name: Perform CodeQL Analysis
+ uses: github/codeql-action/analyze@v1
diff --git a/.github/workflows/commands.yml b/.github/workflows/commands.yml
new file mode 100644
index 0000000000000..f61d114b1579f
--- /dev/null
+++ b/.github/workflows/commands.yml
@@ -0,0 +1,24 @@
+name: Commands
+on:
+ issue_comment:
+ types: [created]
+
+# also make changes in ./on-label.yml
+jobs:
+ main:
+ runs-on: ubuntu-latest
+ steps:
+ - name: Checkout Actions
+ uses: actions/checkout@v2
+ with:
+ repository: 'microsoft/vscode-github-triage-actions'
+ path: ./actions
+ ref: v35
+ - name: Install Actions
+ run: npm install --production --prefix ./actions
+ - name: Run Commands
+ uses: ./actions/commands
+ with:
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ token: ${{secrets.VSCODE_ISSUE_TRIAGE_BOT_PAT}}
+ config-path: commands
diff --git a/.github/workflows/deep-classifier-monitor.yml b/.github/workflows/deep-classifier-monitor.yml
new file mode 100644
index 0000000000000..9d4c54dc6be5b
--- /dev/null
+++ b/.github/workflows/deep-classifier-monitor.yml
@@ -0,0 +1,23 @@
+name: "Deep Classifier: Monitor"
+on:
+ issues:
+ types: [unassigned]
+
+jobs:
+ main:
+ runs-on: ubuntu-latest
+ steps:
+ - name: Checkout Actions
+ uses: actions/checkout@v2
+ with:
+ repository: 'microsoft/vscode-github-triage-actions'
+ ref: v35
+ path: ./actions
+ - name: Install Actions
+ run: npm install --production --prefix ./actions
+ - name: "Run Classifier: Monitor"
+ uses: ./actions/classifier-deep/monitor
+ with:
+ botName: vscode-triage-bot
+ token: ${{secrets.VSCODE_ISSUE_TRIAGE_BOT_PAT}}
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
diff --git a/.github/workflows/deep-classifier-runner.yml b/.github/workflows/deep-classifier-runner.yml
new file mode 100644
index 0000000000000..82ae49039fb56
--- /dev/null
+++ b/.github/workflows/deep-classifier-runner.yml
@@ -0,0 +1,50 @@
+name: "Deep Classifier: Runner"
+on:
+ schedule:
+ - cron: 0 * * * *
+ repository_dispatch:
+ types: [trigger-deep-classifier-runner]
+
+jobs:
+ main:
+ runs-on: ubuntu-latest
+ steps:
+ - name: Checkout Actions
+ uses: actions/checkout@v2
+ with:
+ repository: 'microsoft/vscode-github-triage-actions'
+ ref: v35
+ path: ./actions
+ - name: Install Actions
+ run: npm install --production --prefix ./actions
+ - name: Install Additional Dependencies
+ # Pulls in a bunch of other packages that arent needed for the rest of the actions
+ run: npm install @azure/storage-blob@12.1.1
+ - name: "Run Classifier: Scraper"
+ uses: ./actions/classifier-deep/apply/fetch-sources
+ with:
+ # slightly overlapping to protect against issues slipping through the cracks if a run is delayed
+ from: 80
+ until: 5
+ configPath: classifier
+ blobContainerName: vscode-issue-classifier
+ blobStorageKey: ${{secrets.AZURE_BLOB_STORAGE_CONNECTION_STRING}}
+ token: ${{secrets.VSCODE_ISSUE_TRIAGE_BOT_PAT}}
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ - name: Set up Python 3.7
+ uses: actions/setup-python@v1
+ with:
+ python-version: 3.7
+ - name: Install dependencies
+ run: |
+ python -m pip install --upgrade pip
+ pip install --upgrade numpy scipy scikit-learn joblib nltk simpletransformers torch torchvision
+ - name: "Run Classifier: Generator"
+ run: python ./actions/classifier-deep/apply/generate-labels/main.py
+ - name: "Run Classifier: Labeler"
+ uses: ./actions/classifier-deep/apply/apply-labels
+ with:
+ configPath: classifier
+ allowLabels: "needs more info|new release"
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ token: ${{secrets.VSCODE_ISSUE_TRIAGE_BOT_PAT}}
diff --git a/.github/workflows/deep-classifier-scraper.yml b/.github/workflows/deep-classifier-scraper.yml
new file mode 100644
index 0000000000000..9fe52cd3477f8
--- /dev/null
+++ b/.github/workflows/deep-classifier-scraper.yml
@@ -0,0 +1,27 @@
+name: "Deep Classifier: Scraper"
+on:
+ repository_dispatch:
+ types: [trigger-deep-classifier-scraper]
+
+jobs:
+ main:
+ runs-on: ubuntu-latest
+ steps:
+ - name: Checkout Actions
+ uses: actions/checkout@v2
+ with:
+ repository: 'microsoft/vscode-github-triage-actions'
+ ref: v35
+ path: ./actions
+ - name: Install Actions
+ run: npm install --production --prefix ./actions
+ - name: Install Additional Dependencies
+ # Pulls in a bunch of other packages that arent needed for the rest of the actions
+ run: npm install @azure/storage-blob@12.1.1
+ - name: "Run Classifier: Scraper"
+ uses: ./actions/classifier-deep/train/fetch-issues
+ with:
+ blobContainerName: vscode-issue-classifier
+ blobStorageKey: ${{secrets.AZURE_BLOB_STORAGE_CONNECTION_STRING}}
+ token: ${{secrets.ISSUE_SCRAPER_TOKEN}}
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
diff --git a/.github/workflows/english-please.yml b/.github/workflows/english-please.yml
new file mode 100644
index 0000000000000..6cf4d65455cc6
--- /dev/null
+++ b/.github/workflows/english-please.yml
@@ -0,0 +1,31 @@
+name: English Please
+on:
+ issues:
+ types: [edited]
+
+# also make changes in ./on-label.yml and ./on-open.yml
+jobs:
+ main:
+ runs-on: ubuntu-latest
+ steps:
+ - name: Checkout Actions
+ if: contains(github.event.issue.labels.*.name, '*english-please')
+ uses: actions/checkout@v2
+ with:
+ repository: 'microsoft/vscode-github-triage-actions'
+ ref: v35
+ path: ./actions
+ - name: Install Actions
+ if: contains(github.event.issue.labels.*.name, '*english-please')
+ run: npm install --production --prefix ./actions
+ - name: Run English Please
+ if: contains(github.event.issue.labels.*.name, '*english-please')
+ uses: ./actions/english-please
+ with:
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ token: ${{secrets.VSCODE_ISSUE_TRIAGE_BOT_PAT}}
+ cognitiveServicesAPIKey: ${{secrets.AZURE_TEXT_TRANSLATOR_KEY}}
+ nonEnglishLabel: "*english-please"
+ needsMoreInfoLabel: "needs more info"
+ translatorRequestedLabelPrefix: "translation-required-"
+ translatorRequestedLabelColor: "c29cff"
diff --git a/.github/workflows/feature-request.yml b/.github/workflows/feature-request.yml
new file mode 100644
index 0000000000000..6cdf0bd6fc3f7
--- /dev/null
+++ b/.github/workflows/feature-request.yml
@@ -0,0 +1,43 @@
+name: Feature Request Manager
+on:
+ repository_dispatch:
+ types: [trigger-feature-request-manager]
+ issues:
+ types: [milestoned]
+ schedule:
+ - cron: 20 2 * * * # 4:20am Zurich
+
+# also make changes in ./on-label.yml
+jobs:
+ main:
+ runs-on: ubuntu-latest
+ steps:
+ - name: Checkout Actions
+ if: github.event_name != 'issues' || contains(github.event.issue.labels.*.name, 'feature-request')
+ uses: actions/checkout@v2
+ with:
+ repository: 'microsoft/vscode-github-triage-actions'
+ path: ./actions
+ ref: v35
+ - name: Install Actions
+ if: github.event_name != 'issues' || contains(github.event.issue.labels.*.name, 'feature-request')
+ run: npm install --production --prefix ./actions
+ - name: Run Feature Request Manager
+ if: github.event_name != 'issues' || contains(github.event.issue.labels.*.name, 'feature-request')
+ uses: ./actions/feature-request
+ with:
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ token: ${{secrets.VSCODE_ISSUE_TRIAGE_BOT_PAT}}
+ candidateMilestoneID: 107
+ candidateMilestoneName: Backlog Candidates
+ backlogMilestoneID: 8
+ featureRequestLabel: feature-request
+ upvotesRequired: 20
+ numCommentsOverride: 20
+ initComment: "This feature request is now a candidate for our backlog. The community has 60 days to [upvote](https://github.com/microsoft/vscode/wiki/Issues-Triaging#up-voting-a-feature-request) the issue. If it receives 20 upvotes we will move it to our backlog. If not, we will close it. To learn more about how we handle feature requests, please see our [documentation](https://aka.ms/vscode-issue-lifecycle).\n\nHappy Coding!"
+ warnComment: "This feature request has not yet received the 20 community [upvotes](https://github.com/microsoft/vscode/wiki/Issues-Triaging#up-voting-a-feature-request) it takes to make to our backlog. 10 days to go. To learn more about how we handle feature requests, please see our [documentation](https://aka.ms/vscode-issue-lifecycle).\n\nHappy Coding!"
+ acceptComment: ":slightly_smiling_face: This feature request received a sufficient number of community upvotes and we moved it to our backlog. To learn more about how we handle feature requests, please see our [documentation](https://aka.ms/vscode-issue-lifecycle).\n\nHappy Coding!"
+ rejectComment: ":slightly_frowning_face: In the last 60 days, this feature request has received less than 20 community upvotes and we closed it. Still a big Thank You to you for taking the time to create this issue! To learn more about how we handle feature requests, please see our [documentation](https://aka.ms/vscode-issue-lifecycle).\n\nHappy Coding!"
+ warnDays: 10
+ closeDays: 60
+ milestoneDelaySeconds: 60
diff --git a/.github/workflows/latest-release-monitor.yml b/.github/workflows/latest-release-monitor.yml
new file mode 100644
index 0000000000000..55855054383d1
--- /dev/null
+++ b/.github/workflows/latest-release-monitor.yml
@@ -0,0 +1,27 @@
+name: Latest Release Monitor
+on:
+ schedule:
+ - cron: 0/5 * * * *
+ repository_dispatch:
+ types: [trigger-latest-release-monitor]
+
+jobs:
+ main:
+ runs-on: ubuntu-latest
+ steps:
+ - name: Checkout Actions
+ uses: actions/checkout@v2
+ with:
+ repository: 'microsoft/vscode-github-triage-actions'
+ path: ./actions
+ ref: v35
+ - name: Install Actions
+ run: npm install --production --prefix ./actions
+ - name: Install Storage Module
+ run: npm install @azure/storage-blob@12.1.1
+ - name: Run Latest Release Monitor
+ uses: ./actions/latest-release-monitor
+ with:
+ storageKey: ${{secrets.AZURE_BLOB_STORAGE_CONNECTION_STRING}}
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ token: ${{secrets.VSCODE_ISSUE_TRIAGE_BOT_PAT}}
diff --git a/.github/workflows/locker.yml b/.github/workflows/locker.yml
new file mode 100644
index 0000000000000..2d21b96b8336c
--- /dev/null
+++ b/.github/workflows/locker.yml
@@ -0,0 +1,26 @@
+name: Locker
+on:
+ schedule:
+ - cron: 20 23 * * * # 4:20pm Redmond
+ repository_dispatch:
+ types: [trigger-locker]
+
+jobs:
+ main:
+ runs-on: ubuntu-latest
+ steps:
+ - name: Checkout Actions
+ uses: actions/checkout@v2
+ with:
+ repository: 'microsoft/vscode-github-triage-actions'
+ path: ./actions
+ ref: v35
+ - name: Install Actions
+ run: npm install --production --prefix ./actions
+ - name: Run Locker
+ uses: ./actions/locker
+ with:
+ daysSinceClose: 45
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ daysSinceUpdate: 3
+ ignoredLabel: "*out-of-scope"
diff --git a/.github/workflows/needs-more-info-closer.yml b/.github/workflows/needs-more-info-closer.yml
new file mode 100644
index 0000000000000..dae985ca35ffb
--- /dev/null
+++ b/.github/workflows/needs-more-info-closer.yml
@@ -0,0 +1,30 @@
+name: Needs More Info Closer
+on:
+ schedule:
+ - cron: 20 11 * * * # 4:20am Redmond
+ repository_dispatch:
+ types: [trigger-needs-more-info]
+
+jobs:
+ main:
+ runs-on: ubuntu-latest
+ steps:
+ - name: Checkout Actions
+ uses: actions/checkout@v2
+ with:
+ repository: 'microsoft/vscode-github-triage-actions'
+ path: ./actions
+ ref: v35
+ - name: Install Actions
+ run: npm install --production --prefix ./actions
+ - name: Run Needs More Info Closer
+ uses: ./actions/needs-more-info-closer
+ with:
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ token: ${{secrets.VSCODE_ISSUE_TRIAGE_BOT_PAT}}
+ label: needs more info
+ closeDays: 7
+ additionalTeam: "cleidigh|usernamehw|gjsjohnmurray|IllusionMH"
+ closeComment: "This issue has been closed automatically because it needs more information and has not had recent activity. See also our [issue reporting](https://aka.ms/vscodeissuereporting) guidelines.\n\nHappy Coding!"
+ pingDays: 80
+ pingComment: "Hey @${assignee}, this issue might need further attention.\n\n@${author}, you can help us out by closing this issue if the problem no longer exists, or adding more information."
diff --git a/.github/workflows/on-label.yml b/.github/workflows/on-label.yml
new file mode 100644
index 0000000000000..e51b9ac740fb8
--- /dev/null
+++ b/.github/workflows/on-label.yml
@@ -0,0 +1,93 @@
+name: On Label
+on:
+ issues:
+ types: [labeled]
+
+jobs:
+ main:
+ runs-on: ubuntu-latest
+ steps:
+ - name: Checkout Actions
+ uses: actions/checkout@v2
+ with:
+ repository: 'microsoft/vscode-github-triage-actions'
+ ref: v35
+ path: ./actions
+ - name: Install Actions
+ run: npm install --production --prefix ./actions
+
+ # source of truth in ./author-verified.yml
+ - name: Checkout Repo
+ if: contains(github.event.issue.labels.*.name, 'author-verification-requested')
+ uses: actions/checkout@v2
+ with:
+ path: ./repo
+ fetch-depth: 0
+ - name: Run Author Verified
+ if: contains(github.event.issue.labels.*.name, 'author-verification-requested')
+ uses: ./actions/author-verified
+ with:
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ requestVerificationComment: "This bug has been fixed in to the latest release of [VS Code Insiders](https://code.visualstudio.com/insiders/)!\n\n@${author}, you can help us out by confirming things are working as expected in the latest Insiders release. If things look good, please leave a comment with the text `/verified` to let us know. If not, please ensure you're on version ${commit} of Insiders (today's or later - you can use `Help: About` in the command pallete to check), and leave a comment letting us know what isn't working as expected.\n\nHappy Coding!"
+ pendingReleaseLabel: awaiting-insiders-release
+ authorVerificationRequestedLabel: author-verification-requested
+
+ # source of truth in ./commands.yml
+ - name: Run Commands
+ uses: ./actions/commands
+ with:
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ token: ${{secrets.VSCODE_ISSUE_TRIAGE_BOT_PAT}}
+ config-path: commands
+
+ # only here.
+ - name: Run Subscribers
+ uses: ./actions/topic-subscribe
+ with:
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ token: ${{secrets.VSCODE_ISSUE_TRIAGE_BOT_PAT}}
+ config-path: subscribers
+
+ # source of truth in ./feature-request.yml
+ - name: Run Feature Request Manager
+ if: contains(github.event.issue.labels.*.name, 'feature-request')
+ uses: ./actions/feature-request
+ with:
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ token: ${{secrets.VSCODE_ISSUE_TRIAGE_BOT_PAT}}
+ candidateMilestoneID: 107
+ candidateMilestoneName: Backlog Candidates
+ backlogMilestoneID: 8
+ featureRequestLabel: feature-request
+ upvotesRequired: 20
+ numCommentsOverride: 20
+ initComment: "This feature request is now a candidate for our backlog. The community has 60 days to upvote the issue. If it receives 20 upvotes we will move it to our backlog. If not, we will close it. To learn more about how we handle feature requests, please see our [documentation](https://aka.ms/vscode-issue-lifecycle).\n\nHappy Coding!"
+ warnComment: "This feature request has not yet received the 20 community upvotes it takes to make to our backlog. 10 days to go. To learn more about how we handle feature requests, please see our [documentation](https://aka.ms/vscode-issue-lifecycle).\n\nHappy Coding"
+ acceptComment: ":slightly_smiling_face: This feature request received a sufficient number of community upvotes and we moved it to our backlog. To learn more about how we handle feature requests, please see our [documentation](https://aka.ms/vscode-issue-lifecycle).\n\nHappy Coding!"
+ rejectComment: ":slightly_frowning_face: In the last 60 days, this feature request has received less than 20 community upvotes and we closed it. Still a big Thank You to you for taking the time to create this issue! To learn more about how we handle feature requests, please see our [documentation](https://aka.ms/vscode-issue-lifecycle).\n\nHappy Coding!"
+ warnDays: 10
+ closeDays: 60
+ milestoneDelaySeconds: 60
+
+ # source of truth in ./test-plan-item-validator.yml
+ - name: Run Test Plan Item Validator
+ if: contains(github.event.issue.labels.*.name, 'testplan-item') || contains(github.event.issue.labels.*.name, 'invalid-testplan-item')
+ uses: ./actions/test-plan-item-validator
+ with:
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ label: testplan-item
+ invalidLabel: invalid-testplan-item
+ comment: Invalid test plan item. See errors below and the [test plan item spec](https://github.com/microsoft/vscode/wiki/Writing-Test-Plan-Items) for more information. This comment will go away when the issues are resolved.
+
+ # source of truth in ./english-please.yml
+ - name: Run English Please
+ if: contains(github.event.issue.labels.*.name, '*english-please')
+ uses: ./actions/english-please
+ with:
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ token: ${{secrets.VSCODE_ISSUE_TRIAGE_BOT_PAT}}
+ cognitiveServicesAPIKey: ${{secrets.AZURE_TEXT_TRANSLATOR_KEY}}
+ nonEnglishLabel: "*english-please"
+ needsMoreInfoLabel: "needs more info"
+ translatorRequestedLabelPrefix: "translation-required-"
+ translatorRequestedLabelColor: "c29cff"
diff --git a/.github/workflows/on-open.yml b/.github/workflows/on-open.yml
new file mode 100644
index 0000000000000..e985909b446b9
--- /dev/null
+++ b/.github/workflows/on-open.yml
@@ -0,0 +1,69 @@
+name: On Open
+on:
+ issues:
+ types: [opened]
+
+jobs:
+ main:
+ runs-on: ubuntu-latest
+ steps:
+ - name: Checkout Actions
+ uses: actions/checkout@v2
+ with:
+ repository: 'microsoft/vscode-github-triage-actions'
+ ref: v35
+ path: ./actions
+ - name: Install Actions
+ run: npm install --production --prefix ./actions
+
+ - name: Run CopyCat (JacksonKearl/testissues)
+ uses: ./actions/copycat
+ with:
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ token: ${{secrets.VSCODE_ISSUE_TRIAGE_BOT_PAT}}
+ owner: JacksonKearl
+ repo: testissues
+ - name: Run CopyCat (chrmarti/testissues)
+ uses: ./actions/copycat
+ with:
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ token: ${{secrets.VSCODE_ISSUE_TRIAGE_BOT_PAT}}
+ owner: chrmarti
+ repo: testissues
+
+ - name: Run New Release
+ uses: ./actions/new-release
+ with:
+ label: new release
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ labelColor: "006b75"
+ labelDescription: Issues found in a recent release of VS Code
+ days: 5
+
+ - name: Run Clipboard Labeler
+ uses: ./actions/regex-labeler
+ with:
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ label: "invalid"
+ mustNotMatch: "^We have written the needed data into your clipboard because it was too large to send\\. Please paste\\.$"
+ comment: "It looks like you're using the VS Code Issue Reporter but did not paste the text generated into the created issue. We've closed this issue, please open a new one containing the text we placed in your clipboard.\n\nHappy Coding!"
+
+ - name: Run Clipboard Labeler (Chinese)
+ uses: ./actions/regex-labeler
+ with:
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ label: "invalid"
+ mustNotMatch: "^所需的数据太大,无法直接发送。我们已经将其写入剪贴板,请粘贴。$"
+ comment: "看起来您正在使用 VS Code 问题报告程序,但是没有将生成的文本粘贴到创建的问题中。我们将关闭这个问题,请使用剪贴板中的内容创建一个新的问题。\n\n祝您使用愉快!"
+
+ # source of truth in ./english-please.yml
+ - name: Run English Please
+ uses: ./actions/english-please
+ with:
+ token: ${{secrets.VSCODE_ISSUE_TRIAGE_BOT_PAT}}
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ cognitiveServicesAPIKey: ${{secrets.AZURE_TEXT_TRANSLATOR_KEY}}
+ nonEnglishLabel: "*english-please"
+ needsMoreInfoLabel: "needs more info"
+ translatorRequestedLabelPrefix: "translation-required-"
+ translatorRequestedLabelColor: "c29cff"
diff --git a/.github/workflows/release-pipeline-labeler.yml b/.github/workflows/release-pipeline-labeler.yml
new file mode 100644
index 0000000000000..a52b895e51c5a
--- /dev/null
+++ b/.github/workflows/release-pipeline-labeler.yml
@@ -0,0 +1,32 @@
+name: "Release Pipeline Labeler"
+on:
+ issues:
+ types: [closed, reopened]
+ repository_dispatch:
+ types: [released-insider]
+
+jobs:
+ main:
+ runs-on: ubuntu-latest
+ steps:
+ - name: Checkout Actions
+ uses: actions/checkout@v2
+ with:
+ repository: 'microsoft/vscode-github-triage-actions'
+ ref: v35
+ path: ./actions
+ - name: Checkout Repo
+ if: github.event_name != 'issues'
+ uses: actions/checkout@v2
+ with:
+ path: ./repo
+ fetch-depth: 0
+ - name: Install Actions
+ run: npm install --production --prefix ./actions
+ - name: "Run Release Pipeline Labeler"
+ uses: ./actions/release-pipeline
+ with:
+ token: ${{secrets.VSCODE_ISSUE_TRIAGE_BOT_PAT}}
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ notYetReleasedLabel: unreleased
+ insidersReleasedLabel: insiders-released
diff --git a/.github/workflows/rich-navigation.yml b/.github/workflows/rich-navigation.yml
new file mode 100644
index 0000000000000..38afb0d7f4929
--- /dev/null
+++ b/.github/workflows/rich-navigation.yml
@@ -0,0 +1,21 @@
+name: "Rich Navigation Indexing"
+on:
+ pull_request:
+ push:
+ branches:
+ - master
+
+jobs:
+ richnav:
+ runs-on: windows-latest
+ steps:
+ - uses: actions/checkout@v2
+ - name: Install dependencies
+ run: yarn --frozen-lockfile
+ env:
+ CHILD_CONCURRENCY: 1
+ - uses: microsoft/RichCodeNavIndexer@v0.1
+ with:
+ languages: typescript
+ repo-token: ${{ secrets.GITHUB_TOKEN }}
+ continue-on-error: true
diff --git a/.github/workflows/test-plan-item-validator.yml b/.github/workflows/test-plan-item-validator.yml
new file mode 100644
index 0000000000000..7a173b0e2f321
--- /dev/null
+++ b/.github/workflows/test-plan-item-validator.yml
@@ -0,0 +1,28 @@
+name: Test Plan Item Validator
+on:
+ issues:
+ types: [edited]
+
+# also edit in ./on-label.yml
+jobs:
+ main:
+ runs-on: ubuntu-latest
+ steps:
+ - name: Checkout Actions
+ if: contains(github.event.issue.labels.*.name, 'testplan-item') || contains(github.event.issue.labels.*.name, 'invalid-testplan-item')
+ uses: actions/checkout@v2
+ with:
+ repository: 'microsoft/vscode-github-triage-actions'
+ path: ./actions
+ ref: v35
+ - name: Install Actions
+ if: contains(github.event.issue.labels.*.name, 'testplan-item') || contains(github.event.issue.labels.*.name, 'invalid-testplan-item')
+ run: npm install --production --prefix ./actions
+ - name: Run Test Plan Item Validator
+ if: contains(github.event.issue.labels.*.name, 'testplan-item') || contains(github.event.issue.labels.*.name, 'invalid-testplan-item')
+ uses: ./actions/test-plan-item-validator
+ with:
+ label: testplan-item
+ appInsightsKey: ${{secrets.TRIAGE_ACTIONS_APP_INSIGHTS}}
+ invalidLabel: invalid-testplan-item
+ comment: Invalid test plan item. See errors below and the [test plan item spec](https://github.com/microsoft/vscode/wiki/Writing-Test-Plan-Items) for more information. This comment will go away when the issues are resolved.
diff --git a/.gitignore b/.gitignore
index 160c42ed74bda..0fe46b6eadc4c 100644
--- a/.gitignore
+++ b/.gitignore
@@ -11,6 +11,7 @@ out-editor/
out-editor-src/
out-editor-build/
out-editor-esm/
+out-editor-esm-bundle/
out-editor-min/
out-monaco-editor-core/
out-vscode/
@@ -23,6 +24,7 @@ out-vscode-reh-web-min/
out-vscode-reh-web-pkg/
out-vscode-web/
out-vscode-web-min/
+out-vscode-web-pkg/
src/vs/server
resources/server
build/node_modules
diff --git a/.mailmap b/.mailmap
new file mode 100644
index 0000000000000..d54a71fdf1f45
--- /dev/null
+++ b/.mailmap
@@ -0,0 +1,2 @@
+Eric Amodio Eric Amodio
+Daniel Imms Daniel Imms
diff --git a/.prettierrc.json b/.prettierrc.json
deleted file mode 100644
index 91855cc846d98..0000000000000
--- a/.prettierrc.json
+++ /dev/null
@@ -1,6 +0,0 @@
-{
- "useTabs": true,
- "printWidth": 120,
- "semi": true,
- "singleQuote": true
-}
diff --git a/.vscode/extensions.json b/.vscode/extensions.json
index b4336e7d127d5..2cd0a32b9ce77 100644
--- a/.vscode/extensions.json
+++ b/.vscode/extensions.json
@@ -2,7 +2,6 @@
// See https://go.microsoft.com/fwlink/?LinkId=827846
// for the documentation about the extensions.json format
"recommendations": [
- "ms-vscode.vscode-typescript-tslint-plugin",
"dbaeumer.vscode-eslint",
"EditorConfig.EditorConfig",
"msjsdiag.debugger-for-chrome"
diff --git a/.vscode/launch.json b/.vscode/launch.json
index 25279b09fd314..d37ac78321bac 100644
--- a/.vscode/launch.json
+++ b/.vscode/launch.json
@@ -14,19 +14,25 @@
{
"type": "node",
"request": "attach",
+ "restart": true,
"name": "Attach to Extension Host",
+ "timeout": 30000,
"port": 5870,
- "restart": true,
"outFiles": [
- "${workspaceFolder}/out/**/*.js"
+ "${workspaceFolder}/out/**/*.js",
+ "${workspaceFolder}/extensions/*/out/**/*.js"
]
},
{
- "type": "chrome",
+ "type": "pwa-chrome",
"request": "attach",
"name": "Attach to Shared Process",
+ "timeout": 30000,
"port": 9222,
- "urlFilter": "*"
+ "urlFilter": "*sharedProcess.html*",
+ "presentation": {
+ "hidden": true
+ }
},
{
"type": "node",
@@ -35,7 +41,10 @@
"port": 5876,
"outFiles": [
"${workspaceFolder}/out/**/*.js"
- ]
+ ],
+ "presentation": {
+ "hidden": true,
+ }
},
{
"type": "node",
@@ -50,10 +59,14 @@
"type": "node",
"request": "attach",
"name": "Attach to Main Process",
+ "timeout": 30000,
"port": 5875,
"outFiles": [
"${workspaceFolder}/out/**/*.js"
- ]
+ ],
+ "presentation": {
+ "hidden": true,
+ }
},
{
"type": "extensionHost",
@@ -67,7 +80,29 @@
],
"outFiles": [
"${workspaceFolder}/out/**/*.js"
- ]
+ ],
+ "presentation": {
+ "group": "5_tests",
+ "order": 6
+ }
+ },
+ {
+ "type": "extensionHost",
+ "request": "launch",
+ "name": "VS Code Git Tests",
+ "runtimeExecutable": "${execPath}",
+ "args": [
+ "/tmp/my4g9l",
+ "--extensionDevelopmentPath=${workspaceFolder}/extensions/git",
+ "--extensionTestsPath=${workspaceFolder}/extensions/git/out/test"
+ ],
+ "outFiles": [
+ "${workspaceFolder}/extensions/git/out/**/*.js"
+ ],
+ "presentation": {
+ "group": "5_tests",
+ "order": 6
+ }
},
{
"type": "extensionHost",
@@ -82,7 +117,11 @@
],
"outFiles": [
"${workspaceFolder}/out/**/*.js"
- ]
+ ],
+ "presentation": {
+ "group": "5_tests",
+ "order": 3
+ }
},
{
"type": "extensionHost",
@@ -96,7 +135,11 @@
],
"outFiles": [
"${workspaceFolder}/out/**/*.js"
- ]
+ ],
+ "presentation": {
+ "group": "5_tests",
+ "order": 4
+ }
},
{
"type": "extensionHost",
@@ -110,7 +153,47 @@
],
"outFiles": [
"${workspaceFolder}/out/**/*.js"
- ]
+ ],
+ "presentation": {
+ "group": "5_tests",
+ "order": 5
+ }
+ },
+ {
+ "type": "extensionHost",
+ "request": "launch",
+ "name": "VS Code Notebook Tests",
+ "runtimeExecutable": "${execPath}",
+ "args": [
+ "${workspaceFolder}/extensions/vscode-notebook-tests/test",
+ "--extensionDevelopmentPath=${workspaceFolder}/extensions/vscode-notebook-tests",
+ "--extensionTestsPath=${workspaceFolder}/extensions/vscode-notebook-tests/out"
+ ],
+ "outFiles": [
+ "${workspaceFolder}/out/**/*.js"
+ ],
+ "presentation": {
+ "group": "5_tests",
+ "order": 6
+ }
+ },
+ {
+ "type": "extensionHost",
+ "request": "launch",
+ "name": "VS Code Custom Editor Tests",
+ "runtimeExecutable": "${execPath}",
+ "args": [
+ "${workspaceFolder}/extensions/vscode-custom-editor-tests/test-workspace",
+ "--extensionDevelopmentPath=${workspaceFolder}/extensions/vscode-custom-editor-tests",
+ "--extensionTestsPath=${workspaceFolder}/extensions/vscode-custom-editor-tests/out/test"
+ ],
+ "outFiles": [
+ "${workspaceFolder}/out/**/*.js"
+ ],
+ "presentation": {
+ "group": "5_tests",
+ "order": 6
+ }
},
{
"type": "chrome",
@@ -119,7 +202,7 @@
"port": 9222
},
{
- "type": "chrome",
+ "type": "pwa-chrome",
"request": "launch",
"name": "Launch VS Code",
"windows": {
@@ -131,22 +214,44 @@
"linux": {
"runtimeExecutable": "${workspaceFolder}/scripts/code.sh"
},
+ "port": 9222,
"timeout": 20000,
"env": {
- "VSCODE_EXTHOST_WILL_SEND_SOCKET": null
+ "VSCODE_EXTHOST_WILL_SEND_SOCKET": null,
+ "VSCODE_SKIP_PRELAUNCH": "1"
},
- "breakOnLoad": false,
+ "cleanUp": "wholeBrowser",
"urlFilter": "*workbench.html*",
"runtimeArgs": [
"--inspect=5875",
- "--no-cached-data"
+ "--no-cached-data",
+ ],
+ "webRoot": "${workspaceFolder}",
+ "cascadeTerminateToConfigurations": [
+ "Attach to Extension Host"
],
- "webRoot": "${workspaceFolder}"
+ "userDataDir": false,
+ "pauseForSourceMap": false,
+ "outFiles": [
+ "${workspaceFolder}/out/**/*.js"
+ ],
+ "browserLaunchLocation": "workspace",
+ "preLaunchTask": "Ensure Prelaunch Dependencies",
+ },
+ {
+ "type": "node",
+ "request": "launch",
+ "name": "VS Code (Web)",
+ "program": "${workspaceFolder}/resources/web/code-web.js",
+ "presentation": {
+ "group": "0_vscode",
+ "order": 2
+ }
},
{
"type": "node",
"request": "launch",
- "name": "Launch VS Code (Main Process)",
+ "name": "Main Process",
"runtimeExecutable": "${workspaceFolder}/scripts/code.sh",
"windows": {
"runtimeExecutable": "${workspaceFolder}/scripts/code.bat",
@@ -156,23 +261,34 @@
],
"outFiles": [
"${workspaceFolder}/out/**/*.js"
- ]
+ ],
+ "presentation": {
+ "group": "1_vscode",
+ "order": 1
+ }
},
{
- "type": "node",
+ "type": "chrome",
"request": "launch",
- "name": "Launch VS Code (Web)",
- "runtimeExecutable": "yarn",
- "runtimeArgs": [
- "web"
- ],
+ "name": "VS Code (Web, Chrome)",
+ "url": "http://localhost:8080",
+ "preLaunchTask": "Run web",
+ "presentation": {
+ "group": "0_vscode",
+ "order": 3
+ }
},
{
- "type": "chrome",
+ "type": "pwa-msedge",
"request": "launch",
- "name": "Launch VS Code (Web, Chrome)",
+ "name": "VS Code (Web, Edge)",
"url": "http://localhost:8080",
- "preLaunchTask": "Run web"
+ "pauseForSourceMap": false,
+ "preLaunchTask": "Run web",
+ "presentation": {
+ "group": "0_vscode",
+ "order": 3
+ }
},
{
"type": "node",
@@ -183,7 +299,41 @@
"cwd": "${workspaceFolder}/extensions/git",
"outFiles": [
"${workspaceFolder}/extensions/git/out/**/*.js"
- ]
+ ],
+ "presentation": {
+ "group": "5_tests",
+ "order": 10
+ }
+ },
+ {
+ "type": "node",
+ "request": "launch",
+ "name": "HTML Server Unit Tests",
+ "program": "${workspaceFolder}/extensions/html-language-features/server/test/index.js",
+ "stopOnEntry": false,
+ "cwd": "${workspaceFolder}/extensions/html-language-features/server",
+ "outFiles": [
+ "${workspaceFolder}/extensions/html-language-features/server/out/**/*.js"
+ ],
+ "presentation": {
+ "group": "5_tests",
+ "order": 10
+ }
+ },
+ {
+ "type": "node",
+ "request": "launch",
+ "name": "CSS Server Unit Tests",
+ "program": "${workspaceFolder}/extensions/css-language-features/server/test/index.js",
+ "stopOnEntry": false,
+ "cwd": "${workspaceFolder}/extensions/css-language-features/server",
+ "outFiles": [
+ "${workspaceFolder}/extensions/css-language-features/server/out/**/*.js"
+ ],
+ "presentation": {
+ "group": "5_tests",
+ "order": 10
+ }
},
{
"type": "extensionHost",
@@ -191,13 +341,17 @@
"name": "Markdown Extension Tests",
"runtimeExecutable": "${execPath}",
"args": [
- "${workspaceFolder}/extensions/markdown-language-features/test-fixtures",
+ "${workspaceFolder}/extensions/markdown-language-features/test-workspace",
"--extensionDevelopmentPath=${workspaceFolder}/extensions/markdown-language-features",
"--extensionTestsPath=${workspaceFolder}/extensions/markdown-language-features/out/test"
],
"outFiles": [
"${workspaceFolder}/extensions/markdown-language-features/out/**/*.js"
- ]
+ ],
+ "presentation": {
+ "group": "5_tests",
+ "order": 7
+ }
},
{
"type": "extensionHost",
@@ -205,19 +359,23 @@
"name": "TypeScript Extension Tests",
"runtimeExecutable": "${execPath}",
"args": [
- "${workspaceFolder}/extensions/typescript-language-features/test-fixtures",
+ "${workspaceFolder}/extensions/typescript-language-features/test-workspace",
"--extensionDevelopmentPath=${workspaceFolder}/extensions/typescript-language-features",
"--extensionTestsPath=${workspaceFolder}/extensions/typescript-language-features/out/test"
],
"outFiles": [
"${workspaceFolder}/extensions/typescript-language-features/out/**/*.js"
- ]
+ ],
+ "presentation": {
+ "group": "5_tests",
+ "order": 8
+ }
},
{
"type": "node",
"request": "launch",
"name": "Run Unit Tests",
- "program": "${workspaceFolder}/test/electron/index.js",
+ "program": "${workspaceFolder}/test/unit/electron/index.js",
"runtimeExecutable": "${workspaceFolder}/.build/electron/Code - OSS.app/Contents/MacOS/Electron",
"windows": {
"runtimeExecutable": "${workspaceFolder}/.build/electron/Code - OSS.exe"
@@ -235,6 +393,9 @@
],
"env": {
"MOCHA_COLORS": "true"
+ },
+ "presentation": {
+ "hidden": true
}
},
{
@@ -270,33 +431,52 @@
],
"compounds": [
{
- "name": "Debug VS Code Main, Renderer & Extension Host",
+ "name": "VS Code",
+ "stopAll": true,
"configurations": [
"Launch VS Code",
"Attach to Main Process",
- "Attach to Extension Host"
- ]
+ "Attach to Extension Host",
+ "Attach to Shared Process",
+ ],
+ "preLaunchTask": "Ensure Prelaunch Dependencies",
+ "presentation": {
+ "group": "0_vscode",
+ "order": 1
+ }
},
{
"name": "Search and Renderer processes",
"configurations": [
"Launch VS Code",
"Attach to Search Process"
- ]
+ ],
+ "presentation": {
+ "group": "1_vscode",
+ "order": 4
+ }
},
{
"name": "Renderer and Extension Host processes",
"configurations": [
"Launch VS Code",
"Attach to Extension Host"
- ]
+ ],
+ "presentation": {
+ "group": "1_vscode",
+ "order": 3
+ }
},
{
"name": "Debug Unit Tests",
"configurations": [
"Attach to VS Code",
"Run Unit Tests"
- ]
- },
+ ],
+ "presentation": {
+ "group": "1_vscode",
+ "order": 2
+ }
+ }
]
}
diff --git a/.vscode/notebooks/api.github-issues b/.vscode/notebooks/api.github-issues
new file mode 100644
index 0000000000000..2eb4b6432b395
--- /dev/null
+++ b/.vscode/notebooks/api.github-issues
@@ -0,0 +1,38 @@
+[
+ {
+ "kind": 1,
+ "language": "markdown",
+ "value": "#### Config",
+ "editable": true
+ },
+ {
+ "kind": 2,
+ "language": "github-issues",
+ "value": "$repo=repo:microsoft/vscode\n$milestone=milestone:\"September 2020\"",
+ "editable": true
+ },
+ {
+ "kind": 1,
+ "language": "markdown",
+ "value": "### Finalization",
+ "editable": true
+ },
+ {
+ "kind": 2,
+ "language": "github-issues",
+ "value": "$repo $milestone label:api-finalization",
+ "editable": true
+ },
+ {
+ "kind": 1,
+ "language": "markdown",
+ "value": "### Proposals",
+ "editable": true
+ },
+ {
+ "kind": 2,
+ "language": "github-issues",
+ "value": "$repo $milestone is:open label:api-proposal ",
+ "editable": true
+ }
+]
\ No newline at end of file
diff --git a/.vscode/notebooks/inbox.github-issues b/.vscode/notebooks/inbox.github-issues
new file mode 100644
index 0000000000000..e2e9e8a1cbbe1
--- /dev/null
+++ b/.vscode/notebooks/inbox.github-issues
@@ -0,0 +1,50 @@
+[
+ {
+ "kind": 1,
+ "language": "markdown",
+ "value": "##### `Config`: defines the inbox query",
+ "editable": true
+ },
+ {
+ "kind": 2,
+ "language": "github-issues",
+ "value": "$inbox=repo:microsoft/vscode is:open no:assignee -label:feature-request -label:testplan-item -label:plan-item ",
+ "editable": true
+ },
+ {
+ "kind": 1,
+ "language": "markdown",
+ "value": "## Inbox tracking and Issue triage",
+ "editable": true
+ },
+ {
+ "kind": 1,
+ "language": "markdown",
+ "value": "New issues or pull requests submitted by the community are initially triaged by an [automatic classification bot](https://github.com/microsoft/vscode-github-triage-actions/tree/master/classifier-deep). Issues that the bot does not correctly triage are then triaged by a team member. The team rotates the inbox tracker on a weekly basis.\n\nA [mirror](https://github.com/JacksonKearl/testissues/issues) of the VS Code issue stream is available with details about how the bot classifies issues, including feature-area classifications and confidence ratings. Per-category confidence thresholds and feature-area ownership data is maintained in [.github/classifier.json](https://github.com/microsoft/vscode/blob/master/.github/classifier.json). \n\n💡 The bot is being run through a GitHub action that runs every 30 minutes. Give the bot the opportunity to classify an issue before doing it manually.\n\n### Inbox Tracking\n\nThe inbox tracker is responsible for the [global inbox](https://github.com/Microsoft/vscode/issues?utf8=%E2%9C%93&q=is%3Aopen+no%3Aassignee+-label%3Afeature-request+-label%3Atestplan-item+-label%3Aplan-item) containing all **open issues and pull requests** that\n- are neither **feature requests** nor **test plan items** nor **plan items** and\n- have **no owner assignment**.\n\nThe **inbox tracker** may perform any step described in our [issue triaging documentation](https://github.com/microsoft/vscode/wiki/Issues-Triaging) but its main responsibility is to route issues to the actual feature area owner.\n\nFeature area owners track the **feature area inbox** containing all **open issues and pull requests** that\n- are personally assigned to them and are not assigned to any milestone\n- are labeled with their feature area label and are not assigned to any milestone.\nThis secondary triage may involve any of the steps described in our [issue triaging documentation](https://github.com/microsoft/vscode/wiki/Issues-Triaging) and results in a fully triaged or closed issue.\n\nThe [github triage extension](https://github.com/microsoft/vscode-github-triage-extension) can be used to assist with triaging — it provides a \"Command Palette\"-style list of triaging actions like assignment, labeling, and triggers for various bot actions.",
+ "editable": true
+ },
+ {
+ "kind": 1,
+ "language": "markdown",
+ "value": "## Triage Inbox\n\nAll inbox issues but not those that need more information. These issues need to be triaged, e.g assigned to a user or ask for more information",
+ "editable": true
+ },
+ {
+ "kind": 2,
+ "language": "github-issues",
+ "value": "$inbox -label:\"needs more info\" -label:emmet",
+ "editable": true
+ },
+ {
+ "kind": 1,
+ "language": "markdown",
+ "value": "## Inbox\n\nAll issues that have no assignee and that have neither **feature requests** nor **test plan items** nor **plan items**.",
+ "editable": true
+ },
+ {
+ "kind": 2,
+ "language": "github-issues",
+ "value": "$inbox -label:emmet",
+ "editable": true
+ }
+]
\ No newline at end of file
diff --git a/.vscode/notebooks/my-work.github-issues b/.vscode/notebooks/my-work.github-issues
new file mode 100644
index 0000000000000..a5de65e0bd138
--- /dev/null
+++ b/.vscode/notebooks/my-work.github-issues
@@ -0,0 +1,98 @@
+[
+ {
+ "kind": 1,
+ "language": "markdown",
+ "value": "##### `Config`: This should be changed every month/milestone",
+ "editable": true
+ },
+ {
+ "kind": 2,
+ "language": "github-issues",
+ "value": "// list of repos we work in\n$repos=repo:microsoft/vscode repo:microsoft/vscode-remote-release repo:microsoft/vscode-js-debug repo:microsoft/vscode-pull-request-github repo:microsoft/vscode-github-issue-notebooks\n\n// current milestone name\n$milestone=milestone:\"September 2020\"",
+ "editable": true
+ },
+ {
+ "kind": 1,
+ "language": "github-issues",
+ "value": "## Milestone Work",
+ "editable": true
+ },
+ {
+ "kind": 2,
+ "language": "github-issues",
+ "value": "$repos $milestone assignee:@me is:open",
+ "editable": false
+ },
+ {
+ "kind": 1,
+ "language": "github-issues",
+ "value": "## Bugs, Debt, Features...",
+ "editable": true
+ },
+ {
+ "kind": 1,
+ "language": "markdown",
+ "value": "#### My Bugs",
+ "editable": true
+ },
+ {
+ "kind": 2,
+ "language": "github-issues",
+ "value": "$repos assignee:@me is:open label:bug",
+ "editable": true
+ },
+ {
+ "kind": 1,
+ "language": "markdown",
+ "value": "#### Debt & Engineering",
+ "editable": true
+ },
+ {
+ "kind": 2,
+ "language": "github-issues",
+ "value": "$repos assignee:@me is:open label:debt OR $repos assignee:@me is:open label:engineering",
+ "editable": true
+ },
+ {
+ "kind": 1,
+ "language": "markdown",
+ "value": "#### Performance 🐌 🔜 🏎",
+ "editable": true
+ },
+ {
+ "kind": 2,
+ "language": "github-issues",
+ "value": "$repos assignee:@me is:open label:perf OR $repos assignee:@me is:open label:perf-startup OR $repos assignee:@me is:open label:perf-bloat OR $repos assignee:@me is:open label:freeze-slow-crash-leak",
+ "editable": true
+ },
+ {
+ "kind": 1,
+ "language": "markdown",
+ "value": "#### Feature Requests",
+ "editable": true
+ },
+ {
+ "kind": 2,
+ "language": "github-issues",
+ "value": "$repos assignee:@me is:open label:feature-request milestone:Backlog sort:reactions-+1-desc",
+ "editable": true
+ },
+ {
+ "kind": 2,
+ "language": "github-issues",
+ "value": "$repos assignee:@me is:open milestone:\"Backlog Candidates\"",
+ "editable": false
+ },
+ {
+ "kind": 1,
+ "language": "markdown",
+ "value": "#### Not Actionable",
+ "editable": true
+ },
+ {
+ "kind": 2,
+ "language": "github-issues",
+ "value": "$repos assignee:@me is:open label:\"needs more info\"",
+ "editable": false
+ }
+]
\ No newline at end of file
diff --git a/.vscode/notebooks/verification.github-issues b/.vscode/notebooks/verification.github-issues
new file mode 100644
index 0000000000000..4e65452ea56d9
--- /dev/null
+++ b/.vscode/notebooks/verification.github-issues
@@ -0,0 +1,56 @@
+[
+ {
+ "kind": 1,
+ "language": "markdown",
+ "value": "### Bug Verification Queries\n\nBefore shipping we want to verify _all_ bugs. That means when a bug is fixed we check that the fix actually works. It's always best to start with bugs that you have filed and the proceed with bugs that have been filed from users outside the development team. ",
+ "editable": true
+ },
+ {
+ "kind": 1,
+ "language": "markdown",
+ "value": "#### Config: update list of `repos` and the `milestone`",
+ "editable": true
+ },
+ {
+ "kind": 2,
+ "language": "github-issues",
+ "value": "$repos=repo:microsoft/vscode repo:microsoft/vscode-internalbacklog repo:microsoft/vscode-remote-release repo:microsoft/vscode-js-debug repo:microsoft/vscode-pull-request-github repo:microsoft/vscode-github-issue-notebooks \n$milestone=milestone:\"September 2020\"",
+ "editable": true
+ },
+ {
+ "kind": 1,
+ "language": "markdown",
+ "value": "### Bugs You Filed",
+ "editable": true
+ },
+ {
+ "kind": 2,
+ "language": "github-issues",
+ "value": "$repos $milestone is:closed -assignee:@me label:bug -label:verified -label:*duplicate author:@me",
+ "editable": false
+ },
+ {
+ "kind": 1,
+ "language": "markdown",
+ "value": "### Bugs From Outside",
+ "editable": true
+ },
+ {
+ "kind": 2,
+ "language": "github-issues",
+ "value": "$repos $milestone is:closed -assignee:@me label:bug -label:verified -label:*duplicate -author:@me -assignee:@me label:bug -label:verified -author:@me -author:aeschli -author:alexdima -author:alexr00 -author:bpasero -author:chrisdias -author:chrmarti -author:connor4312 -author:dbaeumer -author:deepak1556 -author:eamodio -author:egamma -author:gregvanl -author:isidorn -author:JacksonKearl -author:joaomoreno -author:jrieken -author:lramos15 -author:lszomoru -author:misolori -author:mjbvz -author:rebornix -author:RMacfarlane -author:roblourens -author:sana-ajani -author:sandy081 -author:sbatten -author:Tyriar -author:weinand",
+ "editable": false
+ },
+ {
+ "kind": 1,
+ "language": "markdown",
+ "value": "### All",
+ "editable": true
+ },
+ {
+ "kind": 2,
+ "language": "github-issues",
+ "value": "$repos $milestone is:closed -assignee:@me label:bug -label:verified -label:*duplicate",
+ "editable": false
+ }
+]
\ No newline at end of file
diff --git a/.vscode/searches/TrustedTypes.code-search b/.vscode/searches/TrustedTypes.code-search
new file mode 100644
index 0000000000000..4b61b3e058545
--- /dev/null
+++ b/.vscode/searches/TrustedTypes.code-search
@@ -0,0 +1,194 @@
+# Query: .innerHTML =
+# Flags: CaseSensitive WordMatch
+# Including: src/vs/**/*.{t,j}s
+# Excluding: *.test.ts
+# ContextLines: 3
+
+22 results - 14 files
+
+src/vs/base/browser/markdownRenderer.ts:
+ 161 const strValue = values[0];
+ 162 const span = element.querySelector(`div[data-code="${id}"]`);
+ 163 if (span) {
+ 164: span.innerHTML = strValue;
+ 165 }
+ 166 }).catch(err => {
+ 167 // ignore
+
+ 243 return true;
+ 244 }
+ 245
+ 246: element.innerHTML = insane(renderedMarkdown, {
+ 247 allowedSchemes,
+ 248 // allowedTags should included everything that markdown renders to.
+ 249 // Since we have our own sanitize function for marked, it's possible we missed some tag so let insane make sure.
+
+src/vs/base/browser/ui/contextview/contextview.ts:
+ 157 this.shadowRootHostElement = DOM.$('.shadow-root-host');
+ 158 this.container.appendChild(this.shadowRootHostElement);
+ 159 this.shadowRoot = this.shadowRootHostElement.attachShadow({ mode: 'open' });
+ 160: this.shadowRoot.innerHTML = `
+ 161
+
+src/vs/code/electron-sandbox/issue/issueReporterMain.ts:
+ 57 const platformClass = platform.isWindows ? 'windows' : platform.isLinux ? 'linux' : 'mac';
+ 58 addClass(document.body, platformClass); // used by our fonts
+ 59
+ 60: document.body.innerHTML = BaseHtml();
+ 61 const issueReporter = new IssueReporter(configuration);
+ 62 issueReporter.render();
+ 63 document.body.style.display = 'block';
+
+src/vs/code/electron-sandbox/processExplorer/processExplorerMain.ts:
+ 320 content.push(`.highest { color: ${styles.highlightForeground}; }`);
+ 321 }
+ 322
+ 323: styleTag.innerHTML = content.join('\n');
+ 324 if (document.head) {
+ 325 document.head.appendChild(styleTag);
+ 326 }
+
+src/vs/editor/browser/view/domLineBreaksComputer.ts:
+ 107 allCharOffsets[i] = tmp[0];
+ 108 allVisibleColumns[i] = tmp[1];
+ 109 }
+ 110: containerDomNode.innerHTML = sb.build();
+ 111
+ 112 containerDomNode.style.position = 'absolute';
+ 113 containerDomNode.style.top = '10000';
+
+src/vs/editor/browser/view/viewLayer.ts:
+ 507 private _finishRenderingNewLines(ctx: IRendererContext, domNodeIsEmpty: boolean, newLinesHTML: string, wasNew: boolean[]): void {
+ 508 const lastChild = this.domNode.lastChild;
+ 509 if (domNodeIsEmpty || !lastChild) {
+ 510: this.domNode.innerHTML = newLinesHTML;
+ 511 } else {
+ 512 lastChild.insertAdjacentHTML('afterend', newLinesHTML);
+ 513 }
+
+ 525 private _finishRenderingInvalidLines(ctx: IRendererContext, invalidLinesHTML: string, wasInvalid: boolean[]): void {
+ 526 const hugeDomNode = document.createElement('div');
+ 527
+ 528: hugeDomNode.innerHTML = invalidLinesHTML;
+ 529
+ 530 for (let i = 0; i < ctx.linesLength; i++) {
+ 531 const line = ctx.lines[i];
+
+src/vs/editor/browser/widget/diffEditorWidget.ts:
+ 2157
+ 2158 let domNode = document.createElement('div');
+ 2159 domNode.className = `view-lines line-delete ${MOUSE_CURSOR_TEXT_CSS_CLASS_NAME}`;
+ 2160: domNode.innerHTML = sb.build();
+ 2161 Configuration.applyFontInfoSlow(domNode, fontInfo);
+ 2162
+ 2163 let marginDomNode = document.createElement('div');
+ 2164 marginDomNode.className = 'inline-deleted-margin-view-zone';
+ 2165: marginDomNode.innerHTML = marginHTML.join('');
+ 2166 Configuration.applyFontInfoSlow(marginDomNode, fontInfo);
+ 2167
+ 2168 return {
+
+src/vs/editor/standalone/browser/colorizer.ts:
+ 40 let text = domNode.firstChild ? domNode.firstChild.nodeValue : '';
+ 41 domNode.className += ' ' + theme;
+ 42 let render = (str: string) => {
+ 43: domNode.innerHTML = str;
+ 44 };
+ 45 return this.colorize(modeService, text || '', mimeType, options).then(render, (err) => console.error(err));
+ 46 }
+
+src/vs/editor/standalone/browser/standaloneThemeServiceImpl.ts:
+ 212 if (!this._globalStyleElement) {
+ 213 this._globalStyleElement = dom.createStyleSheet();
+ 214 this._globalStyleElement.className = 'monaco-colors';
+ 215: this._globalStyleElement.innerHTML = this._css;
+ 216 this._styleElements.push(this._globalStyleElement);
+ 217 }
+ 218 return Disposable.None;
+
+ 221 private _registerShadowDomContainer(domNode: HTMLElement): IDisposable {
+ 222 const styleElement = dom.createStyleSheet(domNode);
+ 223 styleElement.className = 'monaco-colors';
+ 224: styleElement.innerHTML = this._css;
+ 225 this._styleElements.push(styleElement);
+ 226 return {
+ 227 dispose: () => {
+
+ 291 ruleCollector.addRule(generateTokensCSSForColorMap(colorMap));
+ 292
+ 293 this._css = cssRules.join('\n');
+ 294: this._styleElements.forEach(styleElement => styleElement.innerHTML = this._css);
+ 295
+ 296 TokenizationRegistry.setColorMap(colorMap);
+ 297 this._onColorThemeChange.fire(theme);
+
+src/vs/editor/test/browser/controller/imeTester.ts:
+ 55 let content = this._model.getModelLineContent(i);
+ 56 r += content + '
';
+ 57 }
+ 58: output.innerHTML = r;
+ 59 }
+ 60 }
+ 61
+
+ 69 let title = document.createElement('div');
+ 70 title.className = 'title';
+ 71
+ 72: title.innerHTML = description + '. Type ' + inputStr + '';
+ 73 container.appendChild(title);
+ 74
+ 75 let startBtn = document.createElement('button');
+
+src/vs/workbench/contrib/notebook/browser/view/renderers/cellRenderer.ts:
+ 454
+ 455 private getMarkdownDragImage(templateData: MarkdownCellRenderTemplate): HTMLElement {
+ 456 const dragImageContainer = DOM.$('.cell-drag-image.monaco-list-row.focused.markdown-cell-row');
+ 457: dragImageContainer.innerHTML = templateData.container.outerHTML;
+ 458
+ 459 // Remove all rendered content nodes after the
+ 460 const markdownContent = dragImageContainer.querySelector('.cell.markdown')!;
+
+ 611 return null;
+ 612 }
+ 613
+ 614: editorContainer.innerHTML = richEditorText;
+ 615
+ 616 return dragImageContainer;
+ 617 }
+
+src/vs/workbench/contrib/notebook/browser/view/renderers/webviewPreloads.ts:
+ 375 addMouseoverListeners(outputNode, outputId);
+ 376 const content = data.content;
+ 377 if (content.type === RenderOutputType.Html) {
+ 378: outputNode.innerHTML = content.htmlContent;
+ 379 cellOutputContainer.appendChild(outputNode);
+ 380 domEval(outputNode);
+ 381 } else {
+
+src/vs/workbench/contrib/webview/browser/pre/main.js:
+ 386 // apply default styles
+ 387 const defaultStyles = newDocument.createElement('style');
+ 388 defaultStyles.id = '_defaultStyles';
+ 389: defaultStyles.innerHTML = defaultCssRules;
+ 390 newDocument.head.prepend(defaultStyles);
+ 391
+ 392 applyStyles(newDocument, newDocument.body);
+
+src/vs/workbench/contrib/welcome/walkThrough/browser/walkThroughPart.ts:
+ 281
+ 282 const content = model.main.textEditorModel.getValue(EndOfLinePreference.LF);
+ 283 if (!strings.endsWith(input.resource.path, '.md')) {
+ 284: this.content.innerHTML = content;
+ 285 this.updateSizeClasses();
+ 286 this.decorateContent();
+ 287 this.contentDisposables.push(this.keybindingService.onDidUpdateKeybindings(() => this.decorateContent()));
+
+ 303 const innerContent = document.createElement('div');
+ 304 innerContent.classList.add('walkThroughContent'); // only for markdown files
+ 305 const markdown = this.expandMacros(content);
+ 306: innerContent.innerHTML = marked(markdown, { renderer });
+ 307 this.content.appendChild(innerContent);
+ 308
+ 309 model.snippets.forEach((snippet, i) => {
diff --git a/.vscode/searches/es6.code-search b/.vscode/searches/es6.code-search
new file mode 100644
index 0000000000000..1e1bdd94188e3
--- /dev/null
+++ b/.vscode/searches/es6.code-search
@@ -0,0 +1,61 @@
+# Query: @deprecated ES6
+# Flags: CaseSensitive WordMatch
+# ContextLines: 2
+
+12 results - 4 files
+
+src/vs/base/browser/dom.ts:
+ 83 };
+ 84
+ 85: /** @deprecated ES6 - use classList*/
+ 86 export const hasClass: (node: HTMLElement | SVGElement, className: string) => boolean = _classList.hasClass.bind(_classList);
+ 87: /** @deprecated ES6 - use classList*/
+ 88 export const addClass: (node: HTMLElement | SVGElement, className: string) => void = _classList.addClass.bind(_classList);
+ 89: /** @deprecated ES6 - use classList*/
+ 90 export const addClasses: (node: HTMLElement | SVGElement, ...classNames: string[]) => void = _classList.addClasses.bind(_classList);
+ 91: /** @deprecated ES6 - use classList*/
+ 92 export const removeClass: (node: HTMLElement | SVGElement, className: string) => void = _classList.removeClass.bind(_classList);
+ 93: /** @deprecated ES6 - use classList*/
+ 94 export const removeClasses: (node: HTMLElement | SVGElement, ...classNames: string[]) => void = _classList.removeClasses.bind(_classList);
+ 95: /** @deprecated ES6 - use classList*/
+ 96 export const toggleClass: (node: HTMLElement | SVGElement, className: string, shouldHaveIt?: boolean) => void = _classList.toggleClass.bind(_classList);
+ 97
+
+src/vs/base/common/arrays.ts:
+ 401
+ 402 /**
+ 403: * @deprecated ES6: use `Array.find`
+ 404 */
+ 405 export function first(array: ReadonlyArray, fn: (item: T) => boolean, notFoundValue: T): T;
+
+src/vs/base/common/objects.ts:
+ 115
+ 116 /**
+ 117: * @deprecated ES6
+ 118 */
+ 119 export function assign(destination: T): T;
+
+src/vs/base/common/strings.ts:
+ 15
+ 16 /**
+ 17: * @deprecated ES6: use `String.padStart`
+ 18 */
+ 19 export function pad(n: number, l: number, char: string = '0'): string {
+
+ 146
+ 147 /**
+ 148: * @deprecated ES6: use `String.startsWith`
+ 149 */
+ 150 export function startsWith(haystack: string, needle: string): boolean {
+
+ 167
+ 168 /**
+ 169: * @deprecated ES6: use `String.endsWith`
+ 170 */
+ 171 export function endsWith(haystack: string, needle: string): boolean {
+
+ 857
+ 858 /**
+ 859: * @deprecated ES6
+ 860 */
+ 861 export function repeat(s: string, count: number): string {
diff --git a/.vscode/searches/ts36031.code-search b/.vscode/searches/ts36031.code-search
new file mode 100644
index 0000000000000..51071b8840af7
--- /dev/null
+++ b/.vscode/searches/ts36031.code-search
@@ -0,0 +1,53 @@
+# Query: \\w+\\?\\.\\w+![(.[]
+# Flags: RegExp
+# ContextLines: 2
+
+8 results - 4 files
+
+src/vs/base/browser/ui/tree/asyncDataTree.ts:
+ 241 } : () => 'treeitem',
+ 242 isChecked: options.accessibilityProvider!.isChecked ? (e) => {
+ 243: return !!(options.accessibilityProvider?.isChecked!(e.element as T));
+ 244 } : undefined,
+ 245 getAriaLabel(e) {
+
+src/vs/platform/list/browser/listService.ts:
+ 463
+ 464 if (typeof options?.openOnSingleClick !== 'boolean' && options?.configurationService) {
+ 465: this.openOnSingleClick = options?.configurationService!.getValue(openModeSettingKey) !== 'doubleClick';
+ 466 this._register(options?.configurationService.onDidChangeConfiguration(() => {
+ 467: this.openOnSingleClick = options?.configurationService!.getValue(openModeSettingKey) !== 'doubleClick';
+ 468 }));
+ 469 } else {
+
+src/vs/workbench/contrib/notebook/browser/notebookEditorWidget.ts:
+ 1526
+ 1527 await this._ensureActiveKernel();
+ 1528: await this._activeKernel?.cancelNotebookCell!(this._notebookViewModel!.uri, undefined);
+ 1529 }
+ 1530
+
+ 1535
+ 1536 await this._ensureActiveKernel();
+ 1537: await this._activeKernel?.executeNotebookCell!(this._notebookViewModel!.uri, undefined);
+ 1538 }
+ 1539
+
+ 1553
+ 1554 await this._ensureActiveKernel();
+ 1555: await this._activeKernel?.cancelNotebookCell!(this._notebookViewModel!.uri, cell.handle);
+ 1556 }
+ 1557
+
+ 1567
+ 1568 await this._ensureActiveKernel();
+ 1569: await this._activeKernel?.executeNotebookCell!(this._notebookViewModel!.uri, cell.handle);
+ 1570 }
+ 1571
+
+src/vs/workbench/contrib/webview/electron-browser/iframeWebviewElement.ts:
+ 89 .then(() => this._resourceRequestManager.ensureReady())
+ 90 .then(() => {
+ 91: this.element?.contentWindow!.postMessage({ channel, args: data }, '*');
+ 92 });
+ 93 }
diff --git a/.vscode/settings.json b/.vscode/settings.json
index ef1f7370d26e3..9239eae0d4dbf 100644
--- a/.vscode/settings.json
+++ b/.vscode/settings.json
@@ -7,7 +7,8 @@
"**/.DS_Store": true,
"build/**/*.js": {
"when": "$(basename).ts"
- }
+ },
+ "src/vs/server": false
},
"files.associations": {
"cglicenses.json": "jsonc"
@@ -22,7 +23,11 @@
"i18n/**": true,
"extensions/**/out/**": true,
"test/smoke/out/**": true,
- "src/vs/base/test/node/uri.test.data.txt": true
+ "test/automation/out/**": true,
+ "test/integration/browser/out/**": true,
+ "src/vs/base/test/node/uri.test.data.txt": true,
+ "src/vs/workbench/test/browser/api/extHostDocumentData.test.perf-data.ts": true,
+ "src/vs/server": false
},
"lcov.path": [
"./.build/coverage/lcov.info",
@@ -37,6 +42,11 @@
}
}
],
+ "eslint.options": {
+ "rulePaths": [
+ "./build/lib/eslint"
+ ]
+ },
"typescript.tsdk": "node_modules/typescript/lib",
"npm.exclude": "**/extensions/**",
"npm.packageManager": "yarn",
@@ -62,5 +72,9 @@
"msjsdiag.debugger-for-chrome": "workspace"
},
"gulp.autoDetect": "off",
- "files.insertFinalNewline": true
+ "files.insertFinalNewline": true,
+ "[typescript]": {
+ "editor.defaultFormatter": "vscode.typescript-language-features"
+ },
+ "typescript.tsc.autoDetect": "off"
}
diff --git a/.vscode/tasks.json b/.vscode/tasks.json
index d1a697bb41daa..943ae5bd53644 100644
--- a/.vscode/tasks.json
+++ b/.vscode/tasks.json
@@ -3,12 +3,34 @@
"tasks": [
{
"type": "npm",
- "script": "watch",
- "label": "Build VS Code",
- "group": {
- "kind": "build",
- "isDefault": true
+ "script": "watch-clientd",
+ "label": "Build VS Code Core",
+ "isBackground": true,
+ "presentation": {
+ "reveal": "never"
},
+ "problemMatcher": {
+ "owner": "typescript",
+ "applyTo": "closedDocuments",
+ "fileLocation": [
+ "absolute"
+ ],
+ "pattern": {
+ "regexp": "Error: ([^(]+)\\((\\d+|\\d+,\\d+|\\d+,\\d+,\\d+,\\d+)\\): (.*)$",
+ "file": 1,
+ "location": 2,
+ "message": 3
+ },
+ "background": {
+ "beginsPattern": "Starting compilation",
+ "endsPattern": "Finished compilation"
+ }
+ }
+ },
+ {
+ "type": "npm",
+ "script": "watch-extensionsd",
+ "label": "Build VS Code Extensions",
"isBackground": true,
"presentation": {
"reveal": "never"
@@ -31,27 +53,81 @@
}
}
},
+ {
+ "label": "Build VS Code",
+ "dependsOn": [
+ "Build VS Code Core",
+ "Build VS Code Extensions"
+ ],
+ "group": {
+ "kind": "build",
+ "isDefault": true
+ }
+ },
+ {
+ "type": "npm",
+ "script": "kill-watch-clientd",
+ "label": "Kill Build VS Code Core",
+ "group": "build",
+ "presentation": {
+ "reveal": "never"
+ },
+ "problemMatcher": "$tsc"
+ },
{
"type": "npm",
- "script": "strict-initialization-watch",
- "label": "TS - Strict Initialization",
+ "script": "kill-watch-extensionsd",
+ "label": "Kill Build VS Code Extensions",
+ "group": "build",
+ "presentation": {
+ "reveal": "never"
+ },
+ "problemMatcher": "$tsc"
+ },
+ {
+ "label": "Kill Build VS Code",
+ "dependsOn": [
+ "Kill Build VS Code Core",
+ "Kill Build VS Code Extensions"
+ ],
+ "group": "build"
+ },
+ {
+ "type": "npm",
+ "script": "watch-webd",
+ "label": "Build Web Extensions",
+ "group": "build",
"isBackground": true,
"presentation": {
"reveal": "never"
},
"problemMatcher": {
- "base": "$tsc-watch",
- "owner": "typescript-strict-initialization",
- "applyTo": "allDocuments"
+ "owner": "typescript",
+ "applyTo": "closedDocuments",
+ "fileLocation": [
+ "absolute"
+ ],
+ "pattern": {
+ "regexp": "Error: ([^(]+)\\((\\d+|\\d+,\\d+|\\d+,\\d+,\\d+,\\d+)\\): (.*)$",
+ "file": 1,
+ "location": 2,
+ "message": 3
+ },
+ "background": {
+ "beginsPattern": "Starting compilation",
+ "endsPattern": "Finished compilation"
+ }
}
},
{
- "type": "gulp",
- "task": "tslint",
- "label": "Run tslint",
- "problemMatcher": [
- "$tslint5"
- ]
+ "type": "npm",
+ "script": "kill-watch-webd",
+ "label": "Kill Build Web Extensions",
+ "group": "build",
+ "presentation": {
+ "reveal": "never"
+ },
+ "problemMatcher": "$tsc"
},
{
"label": "Run tests",
@@ -87,10 +163,9 @@
},
{
"type": "shell",
- "command": "yarn web -- --no-launch",
+ "command": "yarn web --no-launch",
"label": "Run web",
"isBackground": true,
- // This section to make error go away when launching the debug config
"problemMatcher": {
"pattern": {
"regexp": ""
@@ -104,5 +179,35 @@
"reveal": "never"
}
},
+ {
+ "type": "npm",
+ "script": "eslint",
+ "problemMatcher": {
+ "source": "eslint",
+ "base": "$eslint-stylish"
+ }
+ },
+ {
+ "type": "shell",
+ "command": "node build/lib/preLaunch.js",
+ "label": "Ensure Prelaunch Dependencies",
+ "presentation": {
+ "reveal": "silent"
+ }
+ },
+ {
+ "type": "npm",
+ "script": "tsec-compile-check",
+ "problemMatcher": [
+ {
+ "base": "$tsc",
+ "applyTo": "allDocuments",
+ "owner": "tsec"
+ },
+ ],
+ "group": "build",
+ "label": "npm: tsec-compile-check",
+ "detail": "node_modules/tsec/bin/tsec -p src/tsconfig.json --noEmit"
+ }
]
}
diff --git a/.yarnrc b/.yarnrc
index 4c8b70bf6f6ae..fbf612d0989e4 100644
--- a/.yarnrc
+++ b/.yarnrc
@@ -1,3 +1,3 @@
disturl "https://atom.io/download/electron"
-target "6.1.2"
+target "9.3.0"
runtime "electron"
diff --git a/CONTRIBUTING.md b/CONTRIBUTING.md
index 34703fae665bd..b3eb18c2bc0d6 100644
--- a/CONTRIBUTING.md
+++ b/CONTRIBUTING.md
@@ -51,9 +51,9 @@ The built-in tool for reporting an issue, which you can access by using `Report
Please include the following with each issue:
-* Version of VS Code
+* Version of VS Code
-* Your operating system
+* Your operating system
* List of extensions that you have installed
@@ -87,10 +87,11 @@ Once submitted, your report will go into the [issue tracking](https://github.com
## Automated Issue Management
-We use a bot to help us manage issues. This bot currently:
+We use GitHub Actions to help us manage issues. These Actions and their descriptions can be [viewed here](https://github.com/microsoft/vscode-github-triage-actions). Some examples of what these Actions do are:
* Automatically closes any issue marked `needs-more-info` if there has been no response in the past 7 days.
-* Automatically locks issues 45 days after they are closed.
+* Automatically lock issues 45 days after they are closed.
+* Automatically implement the VS Code [feature request pipeline](https://github.com/microsoft/vscode/wiki/Issues-Triaging#managing-feature-requests).
If you believe the bot got something wrong, please open a new issue and let us know.
diff --git a/LICENSE.txt b/LICENSE.txt
index 69be21bd8ed08..0ac28ee234d23 100644
--- a/LICENSE.txt
+++ b/LICENSE.txt
@@ -2,8 +2,6 @@ MIT License
Copyright (c) 2015 - present Microsoft Corporation
-All rights reserved.
-
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
diff --git a/README.md b/README.md
index 5d39d7e40c616..c095a1d190b69 100644
--- a/README.md
+++ b/README.md
@@ -1,7 +1,5 @@
# Visual Studio Code - Open Source ("Code - OSS")
-
-
-[](https://dev.azure.com/vscode/VSCode/_build/latest?definitionId=12)
+[](https://aka.ms/vscode-builds)
[](https://github.com/Microsoft/vscode/issues?q=is%3Aopen+is%3Aissue+label%3Afeature-request+sort%3Areactions-%2B1-desc)
[](https://github.com/Microsoft/vscode/issues?utf8=✓&q=is%3Aissue+is%3Aopen+label%3Abug)
[](https://gitter.im/Microsoft/vscode)
@@ -22,8 +20,6 @@ This repository ("`Code - OSS`") is where we (Microsoft) develop the [Visual Stu
Visual Studio Code is updated monthly with new features and bug fixes. You can download it for Windows, macOS, and Linux on [Visual Studio Code's website](https://code.visualstudio.com/Download). To get the latest releases every day, install the [Insiders build](https://code.visualstudio.com/insiders).
-
-
## Contributing
There are many ways in which you can participate in the project, for example:
@@ -46,17 +42,26 @@ please see the document [How to Contribute](https://github.com/Microsoft/vscode/
* Ask a question on [Stack Overflow](https://stackoverflow.com/questions/tagged/vscode)
* [Request a new feature](CONTRIBUTING.md)
-* Up vote [popular feature requests](https://github.com/Microsoft/vscode/issues?q=is%3Aopen+is%3Aissue+label%3Afeature-request+sort%3Areactions-%2B1-desc)
+* Upvote [popular feature requests](https://github.com/Microsoft/vscode/issues?q=is%3Aopen+is%3Aissue+label%3Afeature-request+sort%3Areactions-%2B1-desc)
* [File an issue](https://github.com/Microsoft/vscode/issues)
* Follow [@code](https://twitter.com/code) and let us know what you think!
## Related Projects
-Many of the core components and extensions to Code live in their own repositories on GitHub. For example, the [node debug adapter](https://github.com/microsoft/vscode-node-debug) and the [mono debug adapter](https://github.com/microsoft/vscode-mono-debug) have their own repositories. For a complete list, please visit the [Related Projects](https://github.com/Microsoft/vscode/wiki/Related-Projects) page on our [wiki](https://github.com/Microsoft/vscode/wiki).
+Many of the core components and extensions to VS Code live in their own repositories on GitHub. For example, the [node debug adapter](https://github.com/microsoft/vscode-node-debug) and the [mono debug adapter](https://github.com/microsoft/vscode-mono-debug) have their own repositories. For a complete list, please visit the [Related Projects](https://github.com/Microsoft/vscode/wiki/Related-Projects) page on our [wiki](https://github.com/Microsoft/vscode/wiki).
## Bundled Extensions
-Code includes a set of built-in extensions located in the [extensions](extensions) folder, including grammars and snippets for many languages. Extensions that provide rich language support (code completion, Go to Definition) for a language have the suffix `language-features`. For example, the `json` extension provides coloring for `JSON` and the `json-language-features` provides rich language support for `JSON`.
+VS Code includes a set of built-in extensions located in the [extensions](extensions) folder, including grammars and snippets for many languages. Extensions that provide rich language support (code completion, Go to Definition) for a language have the suffix `language-features`. For example, the `json` extension provides coloring for `JSON` and the `json-language-features` provides rich language support for `JSON`.
+
+## Development Container
+
+This repository includes a Visual Studio Code Remote - Containers / Codespaces development container.
+
+- For [Remote - Containers](https://aka.ms/vscode-remote/download/containers), use the **Remote-Containers: Open Repository in Container...** command which creates a Docker volume for better disk I/O on macOS and Windows.
+- For Codespaces, install the [Visual Studio Codespaces](https://aka.ms/vscs-ext-vscode) extension in VS Code, and use the **Codespaces: Create New Codespace** command.
+
+Docker / the Codespace should have at least **4 Cores and 6 GB of RAM (8 GB recommended)** to run full build. See the [development container README](.devcontainer/README.md) for more information.
## Code of Conduct
diff --git a/ThirdPartyNotices.txt b/ThirdPartyNotices.txt
index 440edf6844ba8..4cf2d042f8023 100644
--- a/ThirdPartyNotices.txt
+++ b/ThirdPartyNotices.txt
@@ -7,66 +7,65 @@ This project incorporates components from the projects listed below. The origina
1. atom/language-clojure version 0.22.7 (https://github.com/atom/language-clojure)
2. atom/language-coffee-script version 0.49.3 (https://github.com/atom/language-coffee-script)
-3. atom/language-java version 0.31.3 (https://github.com/atom/language-java)
-4. atom/language-sass version 0.61.4 (https://github.com/atom/language-sass)
+3. atom/language-java version 0.31.4 (https://github.com/atom/language-java)
+4. atom/language-sass version 0.62.1 (https://github.com/atom/language-sass)
5. atom/language-shellscript version 0.26.0 (https://github.com/atom/language-shellscript)
6. atom/language-xml version 0.35.2 (https://github.com/atom/language-xml)
-7. Colorsublime-Themes version 0.1.0 (https://github.com/Colorsublime/Colorsublime-Themes)
-8. daaain/Handlebars version 1.8.0 (https://github.com/daaain/Handlebars)
-9. davidrios/pug-tmbundle (https://github.com/davidrios/pug-tmbundle)
-10. definitelytyped (https://github.com/DefinitelyTyped/DefinitelyTyped)
-11. demyte/language-cshtml version 0.3.0 (https://github.com/demyte/language-cshtml)
-12. Document Object Model version 4.0.0 (https://www.w3.org/DOM/)
-13. dotnet/csharp-tmLanguage version 0.1.0 (https://github.com/dotnet/csharp-tmLanguage)
-14. expand-abbreviation version 0.5.8 (https://github.com/emmetio/expand-abbreviation)
-15. fadeevab/make.tmbundle (https://github.com/fadeevab/make.tmbundle)
-16. freebroccolo/atom-language-swift (https://github.com/freebroccolo/atom-language-swift)
-17. HTML 5.1 W3C Working Draft version 08 October 2015 (http://www.w3.org/TR/2015/WD-html51-20151008/)
-18. Ikuyadeu/vscode-R version 0.5.5 (https://github.com/Ikuyadeu/vscode-R)
-19. insane version 2.6.2 (https://github.com/bevacqua/insane)
-20. Ionic documentation version 1.2.4 (https://github.com/ionic-team/ionic-site)
-21. ionide/ionide-fsgrammar (https://github.com/ionide/ionide-fsgrammar)
-22. jeff-hykin/cpp-textmate-grammar version 1.12.11 (https://github.com/jeff-hykin/cpp-textmate-grammar)
-23. jeff-hykin/cpp-textmate-grammar version 1.14.9 (https://github.com/jeff-hykin/cpp-textmate-grammar)
-24. js-beautify version 1.6.8 (https://github.com/beautify-web/js-beautify)
-25. Jxck/assert version 1.0.0 (https://github.com/Jxck/assert)
-26. language-docker (https://github.com/moby/moby)
-27. language-go version 0.44.3 (https://github.com/atom/language-go)
+7. better-go-syntax version 1.0.0 (https://github.com/jeff-hykin/better-go-syntax/ )
+8. Colorsublime-Themes version 0.1.0 (https://github.com/Colorsublime/Colorsublime-Themes)
+9. daaain/Handlebars version 1.8.0 (https://github.com/daaain/Handlebars)
+10. davidrios/pug-tmbundle (https://github.com/davidrios/pug-tmbundle)
+11. definitelytyped (https://github.com/DefinitelyTyped/DefinitelyTyped)
+12. demyte/language-cshtml version 0.3.0 (https://github.com/demyte/language-cshtml)
+13. Document Object Model version 4.0.0 (https://www.w3.org/DOM/)
+14. dotnet/csharp-tmLanguage version 0.1.0 (https://github.com/dotnet/csharp-tmLanguage)
+15. expand-abbreviation version 0.5.8 (https://github.com/emmetio/expand-abbreviation)
+16. fadeevab/make.tmbundle (https://github.com/fadeevab/make.tmbundle)
+17. freebroccolo/atom-language-swift (https://github.com/freebroccolo/atom-language-swift)
+18. HTML 5.1 W3C Working Draft version 08 October 2015 (http://www.w3.org/TR/2015/WD-html51-20151008/)
+19. Ikuyadeu/vscode-R version 1.3.0 (https://github.com/Ikuyadeu/vscode-R)
+20. insane version 2.6.2 (https://github.com/bevacqua/insane)
+21. Ionic documentation version 1.2.4 (https://github.com/ionic-team/ionic-site)
+22. ionide/ionide-fsgrammar (https://github.com/ionide/ionide-fsgrammar)
+23. jeff-hykin/cpp-textmate-grammar version 1.12.11 (https://github.com/jeff-hykin/cpp-textmate-grammar)
+24. jeff-hykin/cpp-textmate-grammar version 1.14.15 (https://github.com/jeff-hykin/cpp-textmate-grammar)
+25. js-beautify version 1.6.8 (https://github.com/beautify-web/js-beautify)
+26. Jxck/assert version 1.0.0 (https://github.com/Jxck/assert)
+27. language-docker (https://github.com/moby/moby)
28. language-less version 0.34.2 (https://github.com/atom/language-less)
-29. language-php version 0.44.2 (https://github.com/atom/language-php)
+29. language-php version 0.44.5 (https://github.com/atom/language-php)
30. language-rust version 0.4.12 (https://github.com/zargony/atom-language-rust)
31. MagicStack/MagicPython version 1.1.1 (https://github.com/MagicStack/MagicPython)
32. marked version 0.6.2 (https://github.com/markedjs/marked)
33. mdn-data version 1.1.12 (https://github.com/mdn/data)
34. Microsoft/TypeScript-TmLanguage version 0.0.1 (https://github.com/Microsoft/TypeScript-TmLanguage)
35. Microsoft/vscode-JSON.tmLanguage (https://github.com/Microsoft/vscode-JSON.tmLanguage)
-36. Microsoft/vscode-mssql version 1.6.0 (https://github.com/Microsoft/vscode-mssql)
+36. Microsoft/vscode-mssql version 1.9.0 (https://github.com/Microsoft/vscode-mssql)
37. mmims/language-batchfile version 0.7.5 (https://github.com/mmims/language-batchfile)
38. octref/language-css version 0.42.11 (https://github.com/octref/language-css)
39. PowerShell/EditorSyntax version 1.0.0 (https://github.com/PowerShell/EditorSyntax)
-40. promise-polyfill version 8.0.0 (https://github.com/taylorhakes/promise-polyfill)
-41. seti-ui version 0.1.0 (https://github.com/jesseweed/seti-ui)
-42. shaders-tmLanguage version 0.1.0 (https://github.com/tgjones/shaders-tmLanguage)
-43. textmate/asp.vb.net.tmbundle (https://github.com/textmate/asp.vb.net.tmbundle)
-44. textmate/c.tmbundle (https://github.com/textmate/c.tmbundle)
-45. textmate/diff.tmbundle (https://github.com/textmate/diff.tmbundle)
-46. textmate/git.tmbundle (https://github.com/textmate/git.tmbundle)
-47. textmate/groovy.tmbundle (https://github.com/textmate/groovy.tmbundle)
-48. textmate/html.tmbundle (https://github.com/textmate/html.tmbundle)
-49. textmate/ini.tmbundle (https://github.com/textmate/ini.tmbundle)
-50. textmate/javascript.tmbundle (https://github.com/textmate/javascript.tmbundle)
-51. textmate/lua.tmbundle (https://github.com/textmate/lua.tmbundle)
-52. textmate/markdown.tmbundle (https://github.com/textmate/markdown.tmbundle)
-53. textmate/perl.tmbundle (https://github.com/textmate/perl.tmbundle)
-54. textmate/ruby.tmbundle (https://github.com/textmate/ruby.tmbundle)
-55. textmate/yaml.tmbundle (https://github.com/textmate/yaml.tmbundle)
-56. TypeScript-TmLanguage version 0.1.8 (https://github.com/Microsoft/TypeScript-TmLanguage)
-57. TypeScript-TmLanguage version 1.0.0 (https://github.com/Microsoft/TypeScript-TmLanguage)
-58. Unicode version 12.0.0 (http://www.unicode.org/)
-59. vscode-codicons version 0.0.1 (https://github.com/microsoft/vscode-codicons)
-60. vscode-logfile-highlighter version 2.5.0 (https://github.com/emilast/vscode-logfile-highlighter)
-61. vscode-swift version 0.0.1 (https://github.com/owensd/vscode-swift)
-62. Web Background Synchronization (https://github.com/WICG/BackgroundSync)
+40. seti-ui version 0.1.0 (https://github.com/jesseweed/seti-ui)
+41. shaders-tmLanguage version 0.1.0 (https://github.com/tgjones/shaders-tmLanguage)
+42. textmate/asp.vb.net.tmbundle (https://github.com/textmate/asp.vb.net.tmbundle)
+43. textmate/c.tmbundle (https://github.com/textmate/c.tmbundle)
+44. textmate/diff.tmbundle (https://github.com/textmate/diff.tmbundle)
+45. textmate/git.tmbundle (https://github.com/textmate/git.tmbundle)
+46. textmate/groovy.tmbundle (https://github.com/textmate/groovy.tmbundle)
+47. textmate/html.tmbundle (https://github.com/textmate/html.tmbundle)
+48. textmate/ini.tmbundle (https://github.com/textmate/ini.tmbundle)
+49. textmate/javascript.tmbundle (https://github.com/textmate/javascript.tmbundle)
+50. textmate/lua.tmbundle (https://github.com/textmate/lua.tmbundle)
+51. textmate/markdown.tmbundle (https://github.com/textmate/markdown.tmbundle)
+52. textmate/perl.tmbundle (https://github.com/textmate/perl.tmbundle)
+53. textmate/ruby.tmbundle (https://github.com/textmate/ruby.tmbundle)
+54. textmate/yaml.tmbundle (https://github.com/textmate/yaml.tmbundle)
+55. TypeScript-TmLanguage version 0.1.8 (https://github.com/Microsoft/TypeScript-TmLanguage)
+56. TypeScript-TmLanguage version 1.0.0 (https://github.com/Microsoft/TypeScript-TmLanguage)
+57. Unicode version 12.0.0 (https://home.unicode.org/)
+58. vscode-codicons version 0.0.1 (https://github.com/microsoft/vscode-codicons)
+59. vscode-logfile-highlighter version 2.8.0 (https://github.com/emilast/vscode-logfile-highlighter)
+60. vscode-swift version 0.0.1 (https://github.com/owensd/vscode-swift)
+61. Web Background Synchronization (https://github.com/WICG/BackgroundSync)
%% atom/language-clojure NOTICES AND INFORMATION BEGIN HERE
@@ -340,6 +339,32 @@ suitability for any purpose.
=========================================
END OF atom/language-xml NOTICES AND INFORMATION
+%% better-go-syntax NOTICES AND INFORMATION BEGIN HERE
+=========================================
+MIT License
+
+Copyright (c) 2019 Jeff Hykin
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
+=========================================
+END OF better-go-syntax NOTICES AND INFORMATION
+
%% Colorsublime-Themes NOTICES AND INFORMATION BEGIN HERE
=========================================
Copyright (c) 2015 Colorsublime.com
@@ -589,7 +614,7 @@ END OF HTML 5.1 W3C Working Draft NOTICES AND INFORMATION
=========================================
MIT License
-Copyright (c) 2017 Yuki Ueda
+Copyright (c) 2019 Yuki Ueda
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
@@ -987,83 +1012,6 @@ Apache License
=========================================
END OF language-docker NOTICES AND INFORMATION
-%% language-go NOTICES AND INFORMATION BEGIN HERE
-=========================================
-The MIT License (MIT)
-
-Copyright (c) 2014 GitHub Inc.
-
-Permission is hereby granted, free of charge, to any person obtaining
-a copy of this software and associated documentation files (the
-"Software"), to deal in the Software without restriction, including
-without limitation the rights to use, copy, modify, merge, publish,
-distribute, sublicense, and/or sell copies of the Software, and to
-permit persons to whom the Software is furnished to do so, subject to
-the following conditions:
-
-The above copyright notice and this permission notice shall be
-included in all copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
-EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
-MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
-NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
-LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
-OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
-WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
-
-
-This package was derived from a TextMate bundle located at
-https://github.com/rsms/Go.tmbundle and distributed under the following
-license, located in `LICENSE`:
-
-Copyright (c) 2009 Rasmus Andersson
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in
-all copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
-THE SOFTWARE.
-
-
-The Go Template grammar was derived from GoSublime located at
-https://github.com/DisposaBoy/GoSublime and distributed under the following
-license, located in `LICENSE.md`:
-
-Copyright (c) 2012 The GoSublime Authors
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in
-all copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
-THE SOFTWARE.
-=========================================
-END OF language-go NOTICES AND INFORMATION
-
%% language-less NOTICES AND INFORMATION BEGIN HERE
=========================================
The MIT License (MIT)
@@ -1692,10 +1640,10 @@ Copyright (c) Microsoft Corporation
All rights reserved.
MIT License
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the ""Software""), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
-Copyright (c) 2016 Sanjay Nagamangalam
+Copyright (c) 2016 Microsoft
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED *AS IS*, WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
------------------------------------------------ END OF LICENSE ------------------------------------------
+----------------------------------------------- END OF LICENSE -----------------------------------------
=========================================
END OF Microsoft/vscode-mssql NOTICES AND INFORMATION
@@ -1790,33 +1738,6 @@ SOFTWARE.
=========================================
END OF PowerShell/EditorSyntax NOTICES AND INFORMATION
-%% promise-polyfill NOTICES AND INFORMATION BEGIN HERE
-=========================================
-The MIT License (MIT)
-
-Copyright (c) 2014 Taylor Hakes
-Copyright (c) 2014 Forbes Lindesay
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in
-all copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
-THE SOFTWARE.
-=========================================
-END OF promise-polyfill NOTICES AND INFORMATION
-
%% seti-ui NOTICES AND INFORMATION BEGIN HERE
=========================================
Copyright (c) 2014 Jesse Weed
diff --git a/azure-pipelines.yml b/azure-pipelines.yml
index e52afca602874..b2f9a13c2b7b9 100644
--- a/azure-pipelines.yml
+++ b/azure-pipelines.yml
@@ -13,6 +13,6 @@ jobs:
- job: macOS
pool:
- vmImage: macOS 10.13
+ vmImage: macOS-latest
steps:
- - template: build/azure-pipelines/darwin/continuous-build-darwin.yml
\ No newline at end of file
+ - template: build/azure-pipelines/darwin/continuous-build-darwin.yml
diff --git a/build/.cachesalt b/build/.cachesalt
index 339d2d379fa4b..3f2ee542ad5fc 100644
--- a/build/.cachesalt
+++ b/build/.cachesalt
@@ -1 +1 @@
-2019-08-30T20:24:23.714Z
+2020-10-05T20:24:23.714Z
diff --git a/build/.nativeignore b/build/.nativeignore
index a4823bf719cef..0e0eb08b283ad 100644
--- a/build/.nativeignore
+++ b/build/.nativeignore
@@ -19,13 +19,6 @@ vscode-sqlite3/build/**
vscode-sqlite3/src/**
!vscode-sqlite3/build/Release/*.node
-oniguruma/binding.gyp
-oniguruma/build/**
-oniguruma/src/**
-oniguruma/deps/**
-!oniguruma/build/Release/*.node
-!oniguruma/src/*.js
-
windows-mutex/binding.gyp
windows-mutex/build/**
windows-mutex/src/**
@@ -83,13 +76,13 @@ node-pty/deps/**
!node-pty/build/Release/*.dll
!node-pty/build/Release/*.node
-nsfw/binding.gyp
-nsfw/build/**
-nsfw/src/**
-nsfw/openpa/**
-nsfw/includes/**
-!nsfw/build/Release/*.node
-!nsfw/**/*.a
+vscode-nsfw/binding.gyp
+vscode-nsfw/build/**
+vscode-nsfw/src/**
+vscode-nsfw/openpa/**
+vscode-nsfw/includes/**
+!vscode-nsfw/build/Release/*.node
+!vscode-nsfw/**/*.a
vsda/build/**
vsda/ci/**
diff --git a/build/.webignore b/build/.webignore
new file mode 100644
index 0000000000000..1035cb291cc19
--- /dev/null
+++ b/build/.webignore
@@ -0,0 +1,27 @@
+# cleanup rules for web node modules, .gitignore style
+
+**/*.txt
+**/*.json
+**/*.md
+**/*.d.ts
+**/*.js.map
+**/LICENSE
+**/CONTRIBUTORS
+
+jschardet/index.js
+jschardet/src/**
+jschardet/dist/jschardet.js
+
+vscode-textmate/webpack.config.js
+
+xterm/src/**
+
+xterm-addon-search/src/**
+xterm-addon-search/out/**
+xterm-addon-search/fixtures/**
+
+xterm-addon-unicode11/src/**
+xterm-addon-unicode11/out/**
+
+xterm-addon-webgl/src/**
+xterm-addon-webgl/out/**
diff --git a/build/azure-pipelines/common/createAsset.ts b/build/azure-pipelines/common/createAsset.ts
index 619c92a04120e..d7e62629cb847 100644
--- a/build/azure-pipelines/common/createAsset.ts
+++ b/build/azure-pipelines/common/createAsset.ts
@@ -44,15 +44,16 @@ async function doesAssetExist(blobService: azure.BlobService, quality: string, b
return existsResult.exists;
}
-async function uploadBlob(blobService: azure.BlobService, quality: string, blobName: string, file: string): Promise {
+async function uploadBlob(blobService: azure.BlobService, quality: string, blobName: string, filePath: string, fileName: string): Promise {
const blobOptions: azure.BlobService.CreateBlockBlobRequestOptions = {
contentSettings: {
- contentType: mime.lookup(file),
+ contentType: mime.lookup(filePath),
+ contentDisposition: `attachment; filename="${fileName}"`,
cacheControl: 'max-age=31536000, public'
}
};
- await new Promise((c, e) => blobService.createBlockBlobFromLocalFile(quality, blobName, file, blobOptions, err => err ? e(err) : c()));
+ await new Promise((c, e) => blobService.createBlockBlobFromLocalFile(quality, blobName, filePath, blobOptions, err => err ? e(err) : c()));
}
function getEnv(name: string): string {
@@ -66,24 +67,24 @@ function getEnv(name: string): string {
}
async function main(): Promise {
- const [, , platform, type, name, file] = process.argv;
+ const [, , platform, type, fileName, filePath] = process.argv;
const quality = getEnv('VSCODE_QUALITY');
const commit = getEnv('BUILD_SOURCEVERSION');
console.log('Creating asset...');
- const stat = await new Promise((c, e) => fs.stat(file, (err, stat) => err ? e(err) : c(stat)));
+ const stat = await new Promise((c, e) => fs.stat(filePath, (err, stat) => err ? e(err) : c(stat)));
const size = stat.size;
console.log('Size:', size);
- const stream = fs.createReadStream(file);
+ const stream = fs.createReadStream(filePath);
const [sha1hash, sha256hash] = await Promise.all([hashStream('sha1', stream), hashStream('sha256', stream)]);
console.log('SHA1:', sha1hash);
console.log('SHA256:', sha256hash);
- const blobName = commit + '/' + name;
+ const blobName = commit + '/' + fileName;
const storageAccount = process.env['AZURE_STORAGE_ACCOUNT_2']!;
const blobService = azure.createBlobService(storageAccount, process.env['AZURE_STORAGE_ACCESS_KEY_2']!)
@@ -98,7 +99,7 @@ async function main(): Promise {
console.log('Uploading blobs to Azure storage...');
- await uploadBlob(blobService, quality, blobName, file);
+ await uploadBlob(blobService, quality, blobName, filePath, fileName);
console.log('Blobs successfully uploaded.');
diff --git a/build/azure-pipelines/common/extract-telemetry.sh b/build/azure-pipelines/common/extract-telemetry.sh
index 84bbd9c537c17..6436e93c8c1e1 100755
--- a/build/azure-pipelines/common/extract-telemetry.sh
+++ b/build/azure-pipelines/common/extract-telemetry.sh
@@ -10,10 +10,10 @@ git clone --depth 1 https://github.com/Microsoft/vscode-node-debug2.git
git clone --depth 1 https://github.com/Microsoft/vscode-node-debug.git
git clone --depth 1 https://github.com/Microsoft/vscode-html-languageservice.git
git clone --depth 1 https://github.com/Microsoft/vscode-json-languageservice.git
-$BUILD_SOURCESDIRECTORY/build/node_modules/.bin/vscode-telemetry-extractor --sourceDir $BUILD_SOURCESDIRECTORY --excludedDir $BUILD_SOURCESDIRECTORY/extensions --outputDir . --applyEndpoints
-$BUILD_SOURCESDIRECTORY/build/node_modules/.bin/vscode-telemetry-extractor --config $BUILD_SOURCESDIRECTORY/build/azure-pipelines/common/telemetry-config.json -o .
+node $BUILD_SOURCESDIRECTORY/build/node_modules/.bin/vscode-telemetry-extractor --sourceDir $BUILD_SOURCESDIRECTORY --excludedDir $BUILD_SOURCESDIRECTORY/extensions --outputDir . --applyEndpoints
+node $BUILD_SOURCESDIRECTORY/build/node_modules/.bin/vscode-telemetry-extractor --config $BUILD_SOURCESDIRECTORY/build/azure-pipelines/common/telemetry-config.json -o .
mkdir -p $BUILD_SOURCESDIRECTORY/.build/telemetry
mv declarations-resolved.json $BUILD_SOURCESDIRECTORY/.build/telemetry/telemetry-core.json
mv config-resolved.json $BUILD_SOURCESDIRECTORY/.build/telemetry/telemetry-extensions.json
cd ..
-rm -rf extraction
\ No newline at end of file
+rm -rf extraction
diff --git a/build/azure-pipelines/common/publish-webview.ts b/build/azure-pipelines/common/publish-webview.ts
index 143b61bb61abb..b1947ebc024f6 100644
--- a/build/azure-pipelines/common/publish-webview.ts
+++ b/build/azure-pipelines/common/publish-webview.ts
@@ -17,7 +17,7 @@ const fileNames = [
];
async function assertContainer(blobService: azure.BlobService, container: string): Promise {
- await new Promise((c, e) => blobService.createContainerIfNotExists(container, { publicAccessLevel: 'blob' }, err => err ? e(err) : c()));
+ await new Promise((c, e) => blobService.createContainerIfNotExists(container, { publicAccessLevel: 'blob' }, err => err ? e(err) : c()));
}
async function doesBlobExist(blobService: azure.BlobService, container: string, blobName: string): Promise {
@@ -33,7 +33,7 @@ async function uploadBlob(blobService: azure.BlobService, container: string, blo
}
};
- await new Promise((c, e) => blobService.createBlockBlobFromLocalFile(container, blobName, file, blobOptions, err => err ? e(err) : c()));
+ await new Promise((c, e) => blobService.createBlockBlobFromLocalFile(container, blobName, file, blobOptions, err => err ? e(err) : c()));
}
async function publish(commit: string, files: readonly string[]): Promise {
diff --git a/build/azure-pipelines/common/releaseBuild.ts b/build/azure-pipelines/common/releaseBuild.ts
index 13d40a960e5f3..ac49e7b0042a8 100644
--- a/build/azure-pipelines/common/releaseBuild.ts
+++ b/build/azure-pipelines/common/releaseBuild.ts
@@ -17,13 +17,46 @@ function getEnv(name: string): string {
return result;
}
+interface Config {
+ id: string;
+ frozen: boolean;
+}
+
+function createDefaultConfig(quality: string): Config {
+ return {
+ id: quality,
+ frozen: false
+ };
+}
+
+async function getConfig(client: CosmosClient, quality: string): Promise {
+ const query = `SELECT TOP 1 * FROM c WHERE c.id = "${quality}"`;
+
+ const res = await client.database('builds').container('config').items.query(query).fetchAll();
+
+ if (res.resources.length === 0) {
+ return createDefaultConfig(quality);
+ }
+
+ return res.resources[0] as Config;
+}
+
async function main(): Promise {
const commit = getEnv('BUILD_SOURCEVERSION');
const quality = getEnv('VSCODE_QUALITY');
+ const client = new CosmosClient({ endpoint: process.env['AZURE_DOCUMENTDB_ENDPOINT']!, key: process.env['AZURE_DOCUMENTDB_MASTERKEY'] });
+ const config = await getConfig(client, quality);
+
+ console.log('Quality config:', config);
+
+ if (config.frozen) {
+ console.log(`Skipping release because quality ${quality} is frozen.`);
+ return;
+ }
+
console.log(`Releasing build ${commit}...`);
- const client = new CosmosClient({ endpoint: process.env['AZURE_DOCUMENTDB_ENDPOINT']!, key: process.env['AZURE_DOCUMENTDB_MASTERKEY'] });
const scripts = client.database('builds').container(quality).scripts;
await scripts.storedProcedure('releaseBuild').execute('', [commit]);
}
diff --git a/build/azure-pipelines/common/symbols.ts b/build/azure-pipelines/common/symbols.ts
deleted file mode 100644
index 153be4f25b1c2..0000000000000
--- a/build/azure-pipelines/common/symbols.ts
+++ /dev/null
@@ -1,228 +0,0 @@
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-
-'use strict';
-
-import * as request from 'request';
-import { createReadStream, createWriteStream, unlink, mkdir } from 'fs';
-import * as github from 'github-releases';
-import { join } from 'path';
-import { tmpdir } from 'os';
-import { promisify } from 'util';
-
-const BASE_URL = 'https://rink.hockeyapp.net/api/2/';
-const HOCKEY_APP_TOKEN_HEADER = 'X-HockeyAppToken';
-
-export interface IVersions {
- app_versions: IVersion[];
-}
-
-export interface IVersion {
- id: number;
- version: string;
-}
-
-export interface IApplicationAccessor {
- accessToken: string;
- appId: string;
-}
-
-export interface IVersionAccessor extends IApplicationAccessor {
- id: string;
-}
-
-enum Platform {
- WIN_32 = 'win32-ia32',
- WIN_64 = 'win32-x64',
- LINUX_64 = 'linux-x64',
- MAC_OS = 'darwin-x64'
-}
-
-function symbolsZipName(platform: Platform, electronVersion: string, insiders: boolean): string {
- return `${insiders ? 'insiders' : 'stable'}-symbols-v${electronVersion}-${platform}.zip`;
-}
-
-const SEED = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789";
-async function tmpFile(name: string): Promise {
- let res = '';
- for (let i = 0; i < 8; i++) {
- res += SEED.charAt(Math.floor(Math.random() * SEED.length));
- }
-
- const tmpParent = join(tmpdir(), res);
-
- await promisify(mkdir)(tmpParent);
-
- return join(tmpParent, name);
-}
-
-function getVersions(accessor: IApplicationAccessor): Promise {
- return asyncRequest({
- url: `${BASE_URL}/apps/${accessor.appId}/app_versions`,
- method: 'GET',
- headers: {
- [HOCKEY_APP_TOKEN_HEADER]: accessor.accessToken
- }
- });
-}
-
-function createVersion(accessor: IApplicationAccessor, version: string): Promise {
- return asyncRequest({
- url: `${BASE_URL}/apps/${accessor.appId}/app_versions/new`,
- method: 'POST',
- headers: {
- [HOCKEY_APP_TOKEN_HEADER]: accessor.accessToken
- },
- formData: {
- bundle_version: version
- }
- });
-}
-
-function updateVersion(accessor: IVersionAccessor, symbolsPath: string) {
- return asyncRequest({
- url: `${BASE_URL}/apps/${accessor.appId}/app_versions/${accessor.id}`,
- method: 'PUT',
- headers: {
- [HOCKEY_APP_TOKEN_HEADER]: accessor.accessToken
- },
- formData: {
- dsym: createReadStream(symbolsPath)
- }
- });
-}
-
-function asyncRequest(options: request.UrlOptions & request.CoreOptions): Promise {
- return new Promise((resolve, reject) => {
- request(options, (error, _response, body) => {
- if (error) {
- reject(error);
- } else {
- resolve(JSON.parse(body));
- }
- });
- });
-}
-
-function downloadAsset(repository: any, assetName: string, targetPath: string, electronVersion: string) {
- return new Promise((resolve, reject) => {
- repository.getReleases({ tag_name: `v${electronVersion}` }, (err: any, releases: any) => {
- if (err) {
- reject(err);
- } else {
- const asset = releases[0].assets.filter((asset: any) => asset.name === assetName)[0];
- if (!asset) {
- reject(new Error(`Asset with name ${assetName} not found`));
- } else {
- repository.downloadAsset(asset, (err: any, reader: any) => {
- if (err) {
- reject(err);
- } else {
- const writer = createWriteStream(targetPath);
- writer.on('error', reject);
- writer.on('close', resolve);
- reader.on('error', reject);
-
- reader.pipe(writer);
- }
- });
- }
- }
- });
- });
-}
-
-interface IOptions {
- repository: string;
- platform: Platform;
- versions: { code: string; insiders: boolean; electron: string; };
- access: { hockeyAppToken: string; hockeyAppId: string; githubToken: string };
-}
-
-async function ensureVersionAndSymbols(options: IOptions) {
-
- // Check version does not exist
- console.log(`HockeyApp: checking for existing version ${options.versions.code} (${options.platform})`);
- const versions = await getVersions({ accessToken: options.access.hockeyAppToken, appId: options.access.hockeyAppId });
- if (!Array.isArray(versions.app_versions)) {
- throw new Error(`Unexpected response: ${JSON.stringify(versions)}`);
- }
-
- if (versions.app_versions.some(v => v.version === options.versions.code)) {
- console.log(`HockeyApp: Returning without uploading symbols because version ${options.versions.code} (${options.platform}) was already found`);
- return;
- }
-
- // Download symbols for platform and electron version
- const symbolsName = symbolsZipName(options.platform, options.versions.electron, options.versions.insiders);
- const symbolsPath = await tmpFile('symbols.zip');
- console.log(`HockeyApp: downloading symbols ${symbolsName} for electron ${options.versions.electron} (${options.platform}) into ${symbolsPath}`);
- await downloadAsset(new (github as any)({ repo: options.repository, token: options.access.githubToken }), symbolsName, symbolsPath, options.versions.electron);
-
- // Create version
- console.log(`HockeyApp: creating new version ${options.versions.code} (${options.platform})`);
- const version = await createVersion({ accessToken: options.access.hockeyAppToken, appId: options.access.hockeyAppId }, options.versions.code);
-
- // Upload symbols
- console.log(`HockeyApp: uploading symbols for version ${options.versions.code} (${options.platform})`);
- await updateVersion({ id: String(version.id), accessToken: options.access.hockeyAppToken, appId: options.access.hockeyAppId }, symbolsPath);
-
- // Cleanup
- await promisify(unlink)(symbolsPath);
-}
-
-// Environment
-const pakage = require('../../../package.json');
-const product = require('../../../product.json');
-const repository = product.electronRepository;
-const electronVersion = require('../../lib/electron').getElectronVersion();
-const insiders = product.quality !== 'stable';
-let codeVersion = pakage.version;
-if (insiders) {
- codeVersion = `${codeVersion}-insider`;
-}
-const githubToken = process.argv[2];
-const hockeyAppToken = process.argv[3];
-const is64 = process.argv[4] === 'x64';
-const hockeyAppId = process.argv[5];
-
-if (process.argv.length !== 6) {
- throw new Error(`HockeyApp: Unexpected number of arguments. Got ${process.argv}`);
-}
-
-let platform: Platform;
-if (process.platform === 'darwin') {
- platform = Platform.MAC_OS;
-} else if (process.platform === 'win32') {
- platform = is64 ? Platform.WIN_64 : Platform.WIN_32;
-} else {
- platform = Platform.LINUX_64;
-}
-
-// Create version and upload symbols in HockeyApp
-if (repository && codeVersion && electronVersion && (product.quality === 'stable' || product.quality === 'insider')) {
- ensureVersionAndSymbols({
- repository,
- platform,
- versions: {
- code: codeVersion,
- insiders,
- electron: electronVersion
- },
- access: {
- githubToken,
- hockeyAppToken,
- hockeyAppId
- }
- }).then(() => {
- console.log('HockeyApp: done');
- }).catch(error => {
- console.error(`HockeyApp: error ${error} (AppID: ${hockeyAppId})`);
-
- return process.exit(1);
- });
-} else {
- console.log(`HockeyApp: skipping due to unexpected context (repository: ${repository}, codeVersion: ${codeVersion}, electronVersion: ${electronVersion}, quality: ${product.quality})`);
-}
\ No newline at end of file
diff --git a/build/azure-pipelines/darwin/app-entitlements.plist b/build/azure-pipelines/darwin/app-entitlements.plist
new file mode 100644
index 0000000000000..90031d937be48
--- /dev/null
+++ b/build/azure-pipelines/darwin/app-entitlements.plist
@@ -0,0 +1,12 @@
+
+
+
+
+ com.apple.security.cs.allow-jit
+
+ com.apple.security.cs.allow-unsigned-executable-memory
+
+ com.apple.security.cs.allow-dyld-environment-variables
+
+
+
diff --git a/build/azure-pipelines/darwin/continuous-build-darwin.yml b/build/azure-pipelines/darwin/continuous-build-darwin.yml
index 476ee9137a1bd..631b9af7f1068 100644
--- a/build/azure-pipelines/darwin/continuous-build-darwin.yml
+++ b/build/azure-pipelines/darwin/continuous-build-darwin.yml
@@ -1,49 +1,70 @@
steps:
- task: NodeTool@0
inputs:
- versionSpec: "12.13.0"
+ versionSpec: "12.14.1"
+
+- task: geeklearningio.gl-vsts-tasks-yarn.yarn-installer-task.YarnInstaller@2
+ inputs:
+ versionSpec: "1.x"
+
- task: 1ESLighthouseEng.PipelineArtifactCaching.RestoreCacheV1.RestoreCache@1
inputs:
keyfile: '.yarnrc, remote/.yarnrc, **/yarn.lock, !**/node_modules/**/yarn.lock, !**/.*/**/yarn.lock'
targetfolder: '**/node_modules, !**/node_modules/**/node_modules'
- vstsFeed: '$(ArtifactFeed)'
-- task: geeklearningio.gl-vsts-tasks-yarn.yarn-installer-task.YarnInstaller@2
- inputs:
- versionSpec: "1.x"
+ vstsFeed: 'vscode-build-cache'
+
- script: |
CHILD_CONCURRENCY=1 yarn --frozen-lockfile
displayName: Install Dependencies
condition: and(succeeded(), ne(variables['CacheRestored'], 'true'))
+
- task: 1ESLighthouseEng.PipelineArtifactCaching.SaveCacheV1.SaveCache@1
inputs:
keyfile: '.yarnrc, remote/.yarnrc, **/yarn.lock, !**/node_modules/**/yarn.lock, !**/.*/**/yarn.lock'
targetfolder: '**/node_modules, !**/node_modules/**/node_modules'
- vstsFeed: '$(ArtifactFeed)'
+ vstsFeed: 'vscode-build-cache'
condition: and(succeeded(), ne(variables['CacheRestored'], 'true'))
+
- script: |
yarn electron x64
displayName: Download Electron
-- script: |
- yarn gulp hygiene --skip-tslint
- displayName: Run Hygiene Checks
-- script: |
- yarn gulp tslint
- displayName: Run TSLint Checks
+
- script: |
yarn monaco-compile-check
displayName: Run Monaco Editor Checks
+
+- script: |
+ yarn valid-layers-check
+ displayName: Run Valid Layers Checks
+
- script: |
yarn compile
displayName: Compile Sources
+
- script: |
yarn download-builtin-extensions
displayName: Download Built-in Extensions
+
- script: |
./scripts/test.sh --tfs "Unit Tests"
- displayName: Run Unit Tests
+ displayName: Run Unit Tests (Electron)
+
+- script: |
+ yarn test-browser --browser chromium --browser webkit --browser firefox --tfs "Browser Unit Tests"
+ displayName: Run Unit Tests (Browser)
+
- script: |
./scripts/test-integration.sh --tfs "Integration Tests"
- displayName: Run Integration Tests
+ displayName: Run Integration Tests (Electron)
+
+- task: PublishPipelineArtifact@0
+ inputs:
+ artifactName: crash-dump-macos
+ targetPath: .build/crashes
+ displayName: 'Publish Crash Reports'
+ continueOnError: true
+ condition: failed()
+
- task: PublishTestResults@2
displayName: Publish Tests Results
inputs:
diff --git a/build/azure-pipelines/darwin/helper-gpu-entitlements.plist b/build/azure-pipelines/darwin/helper-gpu-entitlements.plist
new file mode 100644
index 0000000000000..4efe1ce508f85
--- /dev/null
+++ b/build/azure-pipelines/darwin/helper-gpu-entitlements.plist
@@ -0,0 +1,8 @@
+
+
+
+
+ com.apple.security.cs.allow-jit
+
+
+
diff --git a/build/azure-pipelines/darwin/helper-plugin-entitlements.plist b/build/azure-pipelines/darwin/helper-plugin-entitlements.plist
new file mode 100644
index 0000000000000..7cd9df032bd08
--- /dev/null
+++ b/build/azure-pipelines/darwin/helper-plugin-entitlements.plist
@@ -0,0 +1,10 @@
+
+
+
+
+ com.apple.security.cs.allow-unsigned-executable-memory
+
+ com.apple.security.cs.disable-library-validation
+
+
+
diff --git a/build/azure-pipelines/darwin/helper-renderer-entitlements.plist b/build/azure-pipelines/darwin/helper-renderer-entitlements.plist
new file mode 100644
index 0000000000000..be8b7163da7fc
--- /dev/null
+++ b/build/azure-pipelines/darwin/helper-renderer-entitlements.plist
@@ -0,0 +1,14 @@
+
+
+
+
+ com.apple.security.cs.allow-jit
+
+ com.apple.security.cs.allow-unsigned-executable-memory
+
+ com.apple.security.cs.disable-library-validation
+
+ com.apple.security.cs.allow-dyld-environment-variables
+
+
+
diff --git a/build/azure-pipelines/darwin/product-build-darwin.yml b/build/azure-pipelines/darwin/product-build-darwin.yml
index 273a728927f25..3b186bb11360c 100644
--- a/build/azure-pipelines/darwin/product-build-darwin.yml
+++ b/build/azure-pipelines/darwin/product-build-darwin.yml
@@ -21,7 +21,7 @@ steps:
- task: NodeTool@0
inputs:
- versionSpec: "12.13.0"
+ versionSpec: "12.14.1"
- task: geeklearningio.gl-vsts-tasks-yarn.yarn-installer-task.YarnInstaller@2
inputs:
@@ -96,9 +96,13 @@ steps:
- script: |
set -e
./scripts/test.sh --build --tfs "Unit Tests"
- # APP_NAME="`ls $(agent.builddirectory)/VSCode-darwin | head -n 1`"
- # yarn smoketest -- --build "$(agent.builddirectory)/VSCode-darwin/$APP_NAME"
- displayName: Run unit tests
+ displayName: Run unit tests (Electron)
+ condition: and(succeeded(), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
+
+- script: |
+ set -e
+ yarn test-browser --build --browser chromium --browser webkit --browser firefox --tfs "Browser Unit Tests"
+ displayName: Run unit tests (Browser)
condition: and(succeeded(), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
- script: |
@@ -111,23 +115,72 @@ steps:
INTEGRATION_TEST_ELECTRON_PATH="$APP_ROOT/$APP_NAME/Contents/MacOS/Electron" \
VSCODE_REMOTE_SERVER_PATH="$(agent.builddirectory)/vscode-reh-darwin" \
./scripts/test-integration.sh --build --tfs "Integration Tests"
- displayName: Run integration tests
+ displayName: Run integration tests (Electron)
condition: and(succeeded(), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
-# Web Smoke Tests disabled due to https://github.com/microsoft/vscode/issues/80308
-# - script: |
-# set -e
-# cd test/smoke
-# yarn compile
-# cd -
-# yarn smoketest --web --headless
-# continueOnError: true
-# displayName: Run web smoke tests
-# condition: and(succeeded(), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
+- script: |
+ set -e
+ VSCODE_REMOTE_SERVER_PATH="$(agent.builddirectory)/vscode-reh-web-darwin" \
+ ./resources/server/test/test-web-integration.sh --browser webkit
+ displayName: Run integration tests (Browser)
+ condition: and(succeeded(), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
- script: |
set -e
- pushd ../VSCode-darwin && zip -r -X -y ../VSCode-darwin.zip * && popd
+ APP_ROOT=$(agent.builddirectory)/VSCode-darwin
+ APP_NAME="`ls $APP_ROOT | head -n 1`"
+ INTEGRATION_TEST_ELECTRON_PATH="$APP_ROOT/$APP_NAME/Contents/MacOS/Electron" \
+ VSCODE_REMOTE_SERVER_PATH="$(agent.builddirectory)/vscode-reh-darwin" \
+ ./resources/server/test/test-remote-integration.sh
+ displayName: Run remote integration tests (Electron)
+ condition: and(succeeded(), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
+
+- script: |
+ set -e
+ APP_ROOT=$(agent.builddirectory)/VSCode-darwin
+ APP_NAME="`ls $APP_ROOT | head -n 1`"
+ yarn smoketest --build "$APP_ROOT/$APP_NAME"
+ continueOnError: true
+ displayName: Run smoke tests (Electron)
+ condition: and(succeeded(), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
+
+- script: |
+ set -e
+ VSCODE_REMOTE_SERVER_PATH="$(agent.builddirectory)/vscode-reh-web-darwin" \
+ yarn smoketest --web --headless
+ continueOnError: true
+ displayName: Run smoke tests (Browser)
+ condition: and(succeeded(), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
+
+- task: PublishPipelineArtifact@0
+ inputs:
+ artifactName: crash-dump-macos
+ targetPath: .build/crashes
+ displayName: 'Publish Crash Reports'
+ continueOnError: true
+ condition: failed()
+
+- task: PublishTestResults@2
+ displayName: Publish Tests Results
+ inputs:
+ testResultsFiles: '*-results.xml'
+ searchFolder: '$(Build.ArtifactStagingDirectory)/test-results'
+ condition: succeededOrFailed()
+
+- script: |
+ set -e
+ security create-keychain -p pwd $(agent.tempdirectory)/buildagent.keychain
+ security default-keychain -s $(agent.tempdirectory)/buildagent.keychain
+ security unlock-keychain -p pwd $(agent.tempdirectory)/buildagent.keychain
+ echo "$(macos-developer-certificate)" | base64 -D > $(agent.tempdirectory)/cert.p12
+ security import $(agent.tempdirectory)/cert.p12 -k $(agent.tempdirectory)/buildagent.keychain -P "$(macos-developer-certificate-key)" -T /usr/bin/codesign
+ security set-key-partition-list -S apple-tool:,apple:,codesign: -s -k pwd $(agent.tempdirectory)/buildagent.keychain
+ DEBUG=electron-osx-sign* node build/darwin/sign.js
+ displayName: Set Hardened Entitlements
+
+- script: |
+ set -e
+ pushd $(agent.builddirectory)/VSCode-darwin && zip -r -X -y $(agent.builddirectory)/VSCode-darwin.zip * && popd
displayName: Archive build
- task: SFP.build-tasks.custom-build-task-1.EsrpCodeSigning@1
@@ -141,24 +194,76 @@ steps:
{
"keyCode": "CP-401337-Apple",
"operationSetCode": "MacAppDeveloperSign",
- "parameters": [ ],
+ "parameters": [
+ {
+ "parameterName": "Hardening",
+ "parameterValue": "--options=runtime"
+ }
+ ],
"toolName": "sign",
"toolVersion": "1.0"
}
]
- SessionTimeout: 120
+ SessionTimeout: 60
displayName: Codesign
+- script: |
+ zip -d $(agent.builddirectory)/VSCode-darwin.zip "*.pkg"
+ displayName: Clean Archive
+
+- script: |
+ APP_ROOT=$(agent.builddirectory)/VSCode-darwin
+ APP_NAME="`ls $APP_ROOT | head -n 1`"
+ BUNDLE_IDENTIFIER=$(node -p "require(\"$APP_ROOT/$APP_NAME/Contents/Resources/app/product.json\").darwinBundleIdentifier")
+ echo "##vso[task.setvariable variable=BundleIdentifier]$BUNDLE_IDENTIFIER"
+ displayName: Export bundle identifier
+
+- task: SFP.build-tasks.custom-build-task-1.EsrpCodeSigning@1
+ inputs:
+ ConnectedServiceName: 'ESRP CodeSign'
+ FolderPath: '$(agent.builddirectory)'
+ Pattern: 'VSCode-darwin.zip'
+ signConfigType: inlineSignParams
+ inlineOperation: |
+ [
+ {
+ "keyCode": "CP-401337-Apple",
+ "operationSetCode": "MacAppNotarize",
+ "parameters": [
+ {
+ "parameterName": "BundleId",
+ "parameterValue": "$(BundleIdentifier)"
+ }
+ ],
+ "toolName": "sign",
+ "toolVersion": "1.0"
+ }
+ ]
+ SessionTimeout: 60
+ displayName: Notarization
+
+- script: |
+ set -e
+ APP_ROOT=$(agent.builddirectory)/VSCode-darwin
+ APP_NAME="`ls $APP_ROOT | head -n 1`"
+ "$APP_ROOT/$APP_NAME/Contents/Resources/app/bin/code" --export-default-configuration=.build
+ displayName: Verify start after signing (export configuration)
+
- script: |
set -e
VSCODE_MIXIN_PASSWORD="$(github-distro-mixin-password)" \
AZURE_DOCUMENTDB_MASTERKEY="$(builds-docdb-key-readwrite)" \
AZURE_STORAGE_ACCESS_KEY="$(ticino-storage-key)" \
AZURE_STORAGE_ACCESS_KEY_2="$(vscode-storage-key)" \
- VSCODE_HOCKEYAPP_TOKEN="$(vscode-hockeyapp-token)" \
./build/azure-pipelines/darwin/publish.sh
displayName: Publish
+- script: |
+ AZURE_STORAGE_ACCESS_KEY="$(ticino-storage-key)" \
+ yarn gulp upload-vscode-configuration
+ displayName: Upload configuration (for Bing settings search)
+ continueOnError: true
+
- task: ms.vss-governance-buildtask.governance-build-task-component-detection.ComponentGovernanceComponentDetection@0
displayName: 'Component Detection'
continueOnError: true
diff --git a/build/azure-pipelines/darwin/publish.sh b/build/azure-pipelines/darwin/publish.sh
index a8067a5eefb96..07734e194f86e 100755
--- a/build/azure-pipelines/darwin/publish.sh
+++ b/build/azure-pipelines/darwin/publish.sh
@@ -1,9 +1,6 @@
#!/usr/bin/env bash
set -e
-# remove pkg from archive
-zip -d ../VSCode-darwin.zip "*.pkg"
-
# publish the build
node build/azure-pipelines/common/createAsset.js \
darwin \
@@ -20,9 +17,3 @@ node build/azure-pipelines/common/createAsset.js \
archive-unsigned \
"vscode-server-darwin.zip" \
../vscode-server-darwin.zip
-
-# publish hockeyapp symbols
-node build/azure-pipelines/common/symbols.js "$VSCODE_MIXIN_PASSWORD" "$VSCODE_HOCKEYAPP_TOKEN" x64 "$VSCODE_HOCKEYAPP_ID_MACOS"
-
-# upload configuration
-yarn gulp upload-vscode-configuration
diff --git a/build/azure-pipelines/distro-build.yml b/build/azure-pipelines/distro-build.yml
index 4689451b54e74..f9bdf7fef8e81 100644
--- a/build/azure-pipelines/distro-build.yml
+++ b/build/azure-pipelines/distro-build.yml
@@ -8,7 +8,7 @@ pr:
steps:
- task: NodeTool@0
inputs:
- versionSpec: "12.13.0"
+ versionSpec: "12.14.1"
- task: AzureKeyVault@1
displayName: 'Azure Key Vault: Get Secrets'
diff --git a/build/azure-pipelines/exploration-build.yml b/build/azure-pipelines/exploration-build.yml
index b91b01138d01b..cf1ced09dc8a5 100644
--- a/build/azure-pipelines/exploration-build.yml
+++ b/build/azure-pipelines/exploration-build.yml
@@ -1,13 +1,17 @@
pool:
vmImage: 'Ubuntu-16.04'
-trigger: none
-pr: none
+trigger:
+ branches:
+ include: ['master']
+pr:
+ branches:
+ include: ['master']
steps:
- task: NodeTool@0
inputs:
- versionSpec: "12.13.0"
+ versionSpec: "12.14.1"
- task: AzureKeyVault@1
displayName: 'Azure Key Vault: Get Secrets'
@@ -27,10 +31,10 @@ steps:
git config user.email "vscode@microsoft.com"
git config user.name "VSCode"
- git checkout origin/electron-6.0.x
+ git checkout origin/electron-11.x.y
git merge origin/master
# Push master branch into exploration branch
- git push origin HEAD:electron-6.0.x
+ git push origin HEAD:electron-11.x.y
displayName: Sync & Merge Exploration
diff --git a/build/azure-pipelines/linux/continuous-build-linux.yml b/build/azure-pipelines/linux/continuous-build-linux.yml
index a87e3753e77a3..41225110f3737 100644
--- a/build/azure-pipelines/linux/continuous-build-linux.yml
+++ b/build/azure-pipelines/linux/continuous-build-linux.yml
@@ -7,51 +7,77 @@ steps:
sudo chmod +x /etc/init.d/xvfb
sudo update-rc.d xvfb defaults
sudo service xvfb start
+
- task: NodeTool@0
inputs:
- versionSpec: "12.13.0"
+ versionSpec: "12.14.1"
+
+- task: geeklearningio.gl-vsts-tasks-yarn.yarn-installer-task.YarnInstaller@2
+ inputs:
+ versionSpec: "1.x"
+
- task: 1ESLighthouseEng.PipelineArtifactCaching.RestoreCacheV1.RestoreCache@1
inputs:
keyfile: '.yarnrc, remote/.yarnrc, **/yarn.lock, !**/node_modules/**/yarn.lock, !**/.*/**/yarn.lock'
targetfolder: '**/node_modules, !**/node_modules/**/node_modules'
- vstsFeed: '$(ArtifactFeed)'
-- task: geeklearningio.gl-vsts-tasks-yarn.yarn-installer-task.YarnInstaller@2
- inputs:
- versionSpec: "1.x"
+ vstsFeed: 'vscode-build-cache'
+
- script: |
CHILD_CONCURRENCY=1 yarn --frozen-lockfile
displayName: Install Dependencies
condition: and(succeeded(), ne(variables['CacheRestored'], 'true'))
+
- task: 1ESLighthouseEng.PipelineArtifactCaching.SaveCacheV1.SaveCache@1
inputs:
keyfile: '.yarnrc, remote/.yarnrc, **/yarn.lock, !**/node_modules/**/yarn.lock, !**/.*/**/yarn.lock'
targetfolder: '**/node_modules, !**/node_modules/**/node_modules'
- vstsFeed: '$(ArtifactFeed)'
+ vstsFeed: 'vscode-build-cache'
condition: and(succeeded(), ne(variables['CacheRestored'], 'true'))
+
- script: |
yarn electron x64
displayName: Download Electron
+
- script: |
- yarn gulp hygiene --skip-tslint
+ yarn gulp hygiene
displayName: Run Hygiene Checks
-- script: |
- yarn gulp tslint
- displayName: Run TSLint Checks
+
- script: |
yarn monaco-compile-check
displayName: Run Monaco Editor Checks
+
+- script: |
+ yarn valid-layers-check
+ displayName: Run Valid Layers Checks
+
- script: |
yarn compile
displayName: Compile Sources
+
- script: |
yarn download-builtin-extensions
displayName: Download Built-in Extensions
+
- script: |
DISPLAY=:10 ./scripts/test.sh --tfs "Unit Tests"
- displayName: Run Unit Tests
+ displayName: Run Unit Tests (Electron)
+
+- script: |
+ DISPLAY=:10 yarn test-browser --browser chromium --tfs "Browser Unit Tests"
+ displayName: Run Unit Tests (Browser)
+
- script: |
DISPLAY=:10 ./scripts/test-integration.sh --tfs "Integration Tests"
- displayName: Run Integration Tests
+ displayName: Run Integration Tests (Electron)
+
+- task: PublishPipelineArtifact@0
+ inputs:
+ artifactName: crash-dump-linux
+ targetPath: .build/crashes
+ displayName: 'Publish Crash Reports'
+ continueOnError: true
+ condition: failed()
+
- task: PublishTestResults@2
displayName: Publish Tests Results
inputs:
diff --git a/build/azure-pipelines/linux/product-build-linux-multiarch.yml b/build/azure-pipelines/linux/product-build-linux-multiarch.yml
index 68ae4ee8b67f9..258f87ea3d2dc 100644
--- a/build/azure-pipelines/linux/product-build-linux-multiarch.yml
+++ b/build/azure-pipelines/linux/product-build-linux-multiarch.yml
@@ -21,7 +21,7 @@ steps:
- task: NodeTool@0
inputs:
- versionSpec: "12.13.0"
+ versionSpec: "12.14.1"
- task: geeklearningio.gl-vsts-tasks-yarn.yarn-installer-task.YarnInstaller@2
inputs:
@@ -107,7 +107,6 @@ steps:
AZURE_DOCUMENTDB_MASTERKEY="$(builds-docdb-key-readwrite)" \
AZURE_STORAGE_ACCESS_KEY_2="$(vscode-storage-key)" \
VSCODE_MIXIN_PASSWORD="$(github-distro-mixin-password)" \
- VSCODE_HOCKEYAPP_TOKEN="$(vscode-hockeyapp-token)" \
./build/azure-pipelines/linux/multiarch/$(VSCODE_ARCH)/publish.sh
displayName: Publish
diff --git a/build/azure-pipelines/linux/product-build-linux.yml b/build/azure-pipelines/linux/product-build-linux.yml
index 6e1f8ec1e536b..270beb898a0fc 100644
--- a/build/azure-pipelines/linux/product-build-linux.yml
+++ b/build/azure-pipelines/linux/product-build-linux.yml
@@ -21,7 +21,7 @@ steps:
- task: NodeTool@0
inputs:
- versionSpec: "12.13.0"
+ versionSpec: "12.14.1"
- task: geeklearningio.gl-vsts-tasks-yarn.yarn-installer-task.YarnInstaller@2
inputs:
@@ -52,21 +52,25 @@ steps:
git merge $(node -p "require('./package.json').distro")
displayName: Merge distro
+- script: |
+ echo -n $VSCODE_ARCH > .build/arch
+ displayName: Prepare arch cache flag
+
- task: 1ESLighthouseEng.PipelineArtifactCaching.RestoreCacheV1.RestoreCache@1
inputs:
- keyfile: 'build/.cachesalt, .yarnrc, remote/.yarnrc, **/yarn.lock, !**/node_modules/**/yarn.lock, !**/.*/**/yarn.lock'
+ keyfile: '.build/arch, build/.cachesalt, .yarnrc, remote/.yarnrc, **/yarn.lock, !**/node_modules/**/yarn.lock, !**/.*/**/yarn.lock'
targetfolder: '**/node_modules, !**/node_modules/**/node_modules'
vstsFeed: 'npm-vscode'
- script: |
set -e
- CHILD_CONCURRENCY=1 yarn --frozen-lockfile
+ CHILD_CONCURRENCY=1 npm_config_arch=$(NPM_ARCH) yarn --frozen-lockfile
displayName: Install dependencies
condition: and(succeeded(), ne(variables['CacheRestored'], 'true'))
- task: 1ESLighthouseEng.PipelineArtifactCaching.SaveCacheV1.SaveCache@1
inputs:
- keyfile: 'build/.cachesalt, .yarnrc, remote/.yarnrc, **/yarn.lock, !**/node_modules/**/yarn.lock, !**/.*/**/yarn.lock'
+ keyfile: '.build/arch, build/.cachesalt, .yarnrc, remote/.yarnrc, **/yarn.lock, !**/node_modules/**/yarn.lock, !**/.*/**/yarn.lock'
targetfolder: '**/node_modules, !**/node_modules/**/node_modules'
vstsFeed: 'npm-vscode'
condition: and(succeeded(), ne(variables['CacheRestored'], 'true'))
@@ -85,53 +89,128 @@ steps:
- script: |
set -e
VSCODE_MIXIN_PASSWORD="$(github-distro-mixin-password)" \
- yarn gulp vscode-linux-x64-min-ci
+ yarn gulp vscode-linux-$(VSCODE_ARCH)-min-ci
VSCODE_MIXIN_PASSWORD="$(github-distro-mixin-password)" \
- yarn gulp vscode-reh-linux-x64-min-ci
+ yarn gulp vscode-reh-linux-$(VSCODE_ARCH)-min-ci
VSCODE_MIXIN_PASSWORD="$(github-distro-mixin-password)" \
- yarn gulp vscode-reh-web-linux-x64-min-ci
+ yarn gulp vscode-reh-web-linux-$(VSCODE_ARCH)-min-ci
displayName: Build
- script: |
set -e
service xvfb start
displayName: Start xvfb
- condition: and(succeeded(), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
+ condition: and(succeeded(), eq(variables['VSCODE_ARCH'], 'x64'), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
- script: |
set -e
DISPLAY=:10 ./scripts/test.sh --build --tfs "Unit Tests"
- displayName: Run unit tests
- condition: and(succeeded(), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
+ displayName: Run unit tests (Electron)
+ condition: and(succeeded(), eq(variables['VSCODE_ARCH'], 'x64'), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
+
+- script: |
+ set -e
+ DISPLAY=:10 yarn test-browser --build --browser chromium --tfs "Browser Unit Tests"
+ displayName: Run unit tests (Browser)
+ condition: and(succeeded(), eq(variables['VSCODE_ARCH'], 'x64'), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
- script: |
# Figure out the full absolute path of the product we just built
# including the remote server and configure the integration tests
# to run with these builds instead of running out of sources.
set -e
- APP_ROOT=$(agent.builddirectory)/VSCode-linux-x64
+ APP_ROOT=$(agent.builddirectory)/VSCode-linux-$(VSCODE_ARCH)
APP_NAME=$(node -p "require(\"$APP_ROOT/resources/app/product.json\").applicationName")
INTEGRATION_TEST_ELECTRON_PATH="$APP_ROOT/$APP_NAME" \
- VSCODE_REMOTE_SERVER_PATH="$(agent.builddirectory)/vscode-reh-linux-x64" \
+ VSCODE_REMOTE_SERVER_PATH="$(agent.builddirectory)/vscode-reh-linux-$(VSCODE_ARCH)" \
DISPLAY=:10 ./scripts/test-integration.sh --build --tfs "Integration Tests"
- # yarn smoketest -- --build "$(agent.builddirectory)/VSCode-linux-x64"
- displayName: Run integration tests
- condition: and(succeeded(), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
+ displayName: Run integration tests (Electron)
+ condition: and(succeeded(), eq(variables['VSCODE_ARCH'], 'x64'), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
+
+- script: |
+ set -e
+ VSCODE_REMOTE_SERVER_PATH="$(agent.builddirectory)/vscode-reh-web-linux-$(VSCODE_ARCH)" \
+ DISPLAY=:10 ./resources/server/test/test-web-integration.sh --browser chromium
+ displayName: Run integration tests (Browser)
+ condition: and(succeeded(), eq(variables['VSCODE_ARCH'], 'x64'), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
+
+- script: |
+ set -e
+ APP_ROOT=$(agent.builddirectory)/VSCode-linux-$(VSCODE_ARCH)
+ APP_NAME=$(node -p "require(\"$APP_ROOT/resources/app/product.json\").applicationName")
+ INTEGRATION_TEST_ELECTRON_PATH="$APP_ROOT/$APP_NAME" \
+ VSCODE_REMOTE_SERVER_PATH="$(agent.builddirectory)/vscode-reh-linux-$(VSCODE_ARCH)" \
+ DISPLAY=:10 ./resources/server/test/test-remote-integration.sh
+ displayName: Run remote integration tests (Electron)
+ condition: and(succeeded(), eq(variables['VSCODE_ARCH'], 'x64'), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
+
+- task: PublishPipelineArtifact@0
+ inputs:
+ artifactName: 'crash-dump-linux-$(VSCODE_ARCH)'
+ targetPath: .build/crashes
+ displayName: 'Publish Crash Reports'
+ continueOnError: true
+ condition: failed()
+
+- task: PublishTestResults@2
+ displayName: Publish Tests Results
+ inputs:
+ testResultsFiles: '*-results.xml'
+ searchFolder: '$(Build.ArtifactStagingDirectory)/test-results'
+ condition: succeededOrFailed()
+
+- script: |
+ set -e
+ yarn gulp "vscode-linux-$(VSCODE_ARCH)-build-deb"
+ yarn gulp "vscode-linux-$(VSCODE_ARCH)-build-rpm"
+ displayName: Build deb, rpm packages
+
+- script: |
+ set -e
+ yarn gulp "vscode-linux-$(VSCODE_ARCH)-prepare-snap"
+ displayName: Prepare snap package
+ condition: and(succeeded(), eq(variables['VSCODE_ARCH'], 'x64'))
+
+# needed for code signing
+- task: UseDotNet@2
+ displayName: 'Install .NET Core SDK 2.x'
+ inputs:
+ version: 2.x
+
+- task: SFP.build-tasks.custom-build-task-1.EsrpCodeSigning@1
+ inputs:
+ ConnectedServiceName: 'ESRP CodeSign'
+ FolderPath: '.build/linux/rpm'
+ Pattern: '*.rpm'
+ signConfigType: inlineSignParams
+ inlineOperation: |
+ [
+ {
+ "keyCode": "CP-450779-Pgp",
+ "operationSetCode": "LinuxSign",
+ "parameters": [ ],
+ "toolName": "sign",
+ "toolVersion": "1.0"
+ }
+ ]
+ SessionTimeout: 120
+ displayName: Codesign rpm
- script: |
set -e
AZURE_DOCUMENTDB_MASTERKEY="$(builds-docdb-key-readwrite)" \
AZURE_STORAGE_ACCESS_KEY_2="$(vscode-storage-key)" \
VSCODE_MIXIN_PASSWORD="$(github-distro-mixin-password)" \
- VSCODE_HOCKEYAPP_TOKEN="$(vscode-hockeyapp-token)" \
+ VSCODE_ARCH="$(VSCODE_ARCH)" \
./build/azure-pipelines/linux/publish.sh
displayName: Publish
- task: PublishPipelineArtifact@0
displayName: 'Publish Pipeline Artifact'
inputs:
- artifactName: snap-x64
+ artifactName: 'snap-$(VSCODE_ARCH)'
targetPath: .build/linux/snap-tarball
+ condition: and(succeeded(), eq(variables['VSCODE_ARCH'], 'x64'))
- task: ms.vss-governance-buildtask.governance-build-task-component-detection.ComponentGovernanceComponentDetection@0
displayName: 'Component Detection'
diff --git a/build/azure-pipelines/linux/publish.sh b/build/azure-pipelines/linux/publish.sh
index 3a5f9683eaac9..72fe2ad7b30e4 100755
--- a/build/azure-pipelines/linux/publish.sh
+++ b/build/azure-pipelines/linux/publish.sh
@@ -4,11 +4,10 @@ REPO="$(pwd)"
ROOT="$REPO/.."
# Publish tarball
-PLATFORM_LINUX="linux-x64"
+PLATFORM_LINUX="linux-$VSCODE_ARCH"
BUILDNAME="VSCode-$PLATFORM_LINUX"
-BUILD="$ROOT/$BUILDNAME"
BUILD_VERSION="$(date +%s)"
-[ -z "$VSCODE_QUALITY" ] && TARBALL_FILENAME="code-$BUILD_VERSION.tar.gz" || TARBALL_FILENAME="code-$VSCODE_QUALITY-$BUILD_VERSION.tar.gz"
+[ -z "$VSCODE_QUALITY" ] && TARBALL_FILENAME="code-$VSCODE_ARCH-$BUILD_VERSION.tar.gz" || TARBALL_FILENAME="code-$VSCODE_QUALITY-$VSCODE_ARCH-$BUILD_VERSION.tar.gz"
TARBALL_PATH="$ROOT/$TARBALL_FILENAME"
rm -rf $ROOT/code-*.tar.*
@@ -27,32 +26,37 @@ rm -rf $ROOT/vscode-server-*.tar.*
node build/azure-pipelines/common/createAsset.js "server-$PLATFORM_LINUX" archive-unsigned "$SERVER_TARBALL_FILENAME" "$SERVER_TARBALL_PATH"
-# Publish hockeyapp symbols
-node build/azure-pipelines/common/symbols.js "$VSCODE_MIXIN_PASSWORD" "$VSCODE_HOCKEYAPP_TOKEN" "x64" "$VSCODE_HOCKEYAPP_ID_LINUX64"
-
# Publish DEB
-yarn gulp "vscode-linux-x64-build-deb"
-PLATFORM_DEB="linux-deb-x64"
-DEB_ARCH="amd64"
+case $VSCODE_ARCH in
+ x64) DEB_ARCH="amd64" ;;
+ *) DEB_ARCH="$VSCODE_ARCH" ;;
+esac
+
+PLATFORM_DEB="linux-deb-$VSCODE_ARCH"
DEB_FILENAME="$(ls $REPO/.build/linux/deb/$DEB_ARCH/deb/)"
DEB_PATH="$REPO/.build/linux/deb/$DEB_ARCH/deb/$DEB_FILENAME"
node build/azure-pipelines/common/createAsset.js "$PLATFORM_DEB" package "$DEB_FILENAME" "$DEB_PATH"
# Publish RPM
-yarn gulp "vscode-linux-x64-build-rpm"
-PLATFORM_RPM="linux-rpm-x64"
-RPM_ARCH="x86_64"
+case $VSCODE_ARCH in
+ x64) RPM_ARCH="x86_64" ;;
+ armhf) RPM_ARCH="armv7hl" ;;
+ arm64) RPM_ARCH="aarch64" ;;
+ *) RPM_ARCH="$VSCODE_ARCH" ;;
+esac
+
+PLATFORM_RPM="linux-rpm-$VSCODE_ARCH"
RPM_FILENAME="$(ls $REPO/.build/linux/rpm/$RPM_ARCH/ | grep .rpm)"
RPM_PATH="$REPO/.build/linux/rpm/$RPM_ARCH/$RPM_FILENAME"
node build/azure-pipelines/common/createAsset.js "$PLATFORM_RPM" package "$RPM_FILENAME" "$RPM_PATH"
-# Publish Snap
-yarn gulp "vscode-linux-x64-prepare-snap"
-
-# Pack snap tarball artifact, in order to preserve file perms
-mkdir -p $REPO/.build/linux/snap-tarball
-SNAP_TARBALL_PATH="$REPO/.build/linux/snap-tarball/snap-x64.tar.gz"
-rm -rf $SNAP_TARBALL_PATH
-(cd .build/linux && tar -czf $SNAP_TARBALL_PATH snap)
+if [ "$VSCODE_ARCH" == "x64" ]; then
+ # Publish Snap
+ # Pack snap tarball artifact, in order to preserve file perms
+ mkdir -p $REPO/.build/linux/snap-tarball
+ SNAP_TARBALL_PATH="$REPO/.build/linux/snap-tarball/snap-$VSCODE_ARCH.tar.gz"
+ rm -rf $SNAP_TARBALL_PATH
+ (cd .build/linux && tar -czf $SNAP_TARBALL_PATH snap)
+fi
diff --git a/build/azure-pipelines/linux/snap-build-linux.yml b/build/azure-pipelines/linux/snap-build-linux.yml
index a530499b31305..39c39e86c9e1d 100644
--- a/build/azure-pipelines/linux/snap-build-linux.yml
+++ b/build/azure-pipelines/linux/snap-build-linux.yml
@@ -1,7 +1,7 @@
steps:
- task: NodeTool@0
inputs:
- versionSpec: "12.13.0"
+ versionSpec: "12.14.1"
- task: geeklearningio.gl-vsts-tasks-yarn.yarn-installer-task.YarnInstaller@2
inputs:
diff --git a/build/azure-pipelines/linux/xvfb.init b/build/azure-pipelines/linux/xvfb.init
index 4d77d253a2649..2365c09f3a460 100644
--- a/build/azure-pipelines/linux/xvfb.init
+++ b/build/azure-pipelines/linux/xvfb.init
@@ -19,7 +19,7 @@
[ "${NETWORKING}" = "no" ] && exit 0
PROG="/usr/bin/Xvfb"
-PROG_OPTIONS=":10 -ac"
+PROG_OPTIONS=":10 -ac -screen 0 1024x768x24"
PROG_OUTPUT="/tmp/Xvfb.out"
case "$1" in
@@ -50,4 +50,4 @@ case "$1" in
exit 1
esac
-exit $RETVAL
\ No newline at end of file
+exit $RETVAL
diff --git a/build/azure-pipelines/mixin.js b/build/azure-pipelines/mixin.js
index efb7d4d1ca94b..e133b2d2bb9f4 100644
--- a/build/azure-pipelines/mixin.js
+++ b/build/azure-pipelines/mixin.js
@@ -12,6 +12,8 @@ const es = require('event-stream');
const vfs = require('vinyl-fs');
const fancyLog = require('fancy-log');
const ansiColors = require('ansi-colors');
+const fs = require('fs');
+const path = require('path');
function main() {
const quality = process.env['VSCODE_QUALITY'];
@@ -21,7 +23,7 @@ function main() {
return;
}
- const productJsonFilter = filter('product.json', { restore: true });
+ const productJsonFilter = filter(f => f.relative === 'product.json', { restore: true });
fancyLog(ansiColors.blue('[mixin]'), `Mixing in sources:`);
return vfs
@@ -29,7 +31,32 @@ function main() {
.pipe(filter(f => !f.isDirectory()))
.pipe(productJsonFilter)
.pipe(buffer())
- .pipe(json(o => Object.assign({}, require('../product.json'), o)))
+ .pipe(json(o => {
+ const ossProduct = JSON.parse(fs.readFileSync(path.join(__dirname, '..', '..', 'product.json'), 'utf8'));
+ let builtInExtensions = ossProduct.builtInExtensions;
+
+ if (Array.isArray(o.builtInExtensions)) {
+ fancyLog(ansiColors.blue('[mixin]'), 'Overwriting built-in extensions:', o.builtInExtensions.map(e => e.name));
+
+ builtInExtensions = o.builtInExtensions;
+ } else if (o.builtInExtensions) {
+ const include = o.builtInExtensions['include'] || [];
+ const exclude = o.builtInExtensions['exclude'] || [];
+
+ fancyLog(ansiColors.blue('[mixin]'), 'OSS built-in extensions:', builtInExtensions.map(e => e.name));
+ fancyLog(ansiColors.blue('[mixin]'), 'Including built-in extensions:', include.map(e => e.name));
+ fancyLog(ansiColors.blue('[mixin]'), 'Excluding built-in extensions:', exclude);
+
+ builtInExtensions = builtInExtensions.filter(ext => !include.find(e => e.name === ext.name) && !exclude.find(name => name === ext.name));
+ builtInExtensions = [...builtInExtensions, ...include];
+
+ fancyLog(ansiColors.blue('[mixin]'), 'Final built-in extensions:', builtInExtensions.map(e => e.name));
+ } else {
+ fancyLog(ansiColors.blue('[mixin]'), 'Inheriting OSS built-in extensions', builtInExtensions.map(e => e.name));
+ }
+
+ return { webBuiltInExtensions: ossProduct.webBuiltInExtensions, ...o, builtInExtensions };
+ }))
.pipe(productJsonFilter.restore)
.pipe(es.mapSync(function (f) {
fancyLog(ansiColors.blue('[mixin]'), f.relative, ansiColors.green('✔︎'));
@@ -38,4 +65,4 @@ function main() {
.pipe(vfs.dest('.'));
}
-main();
\ No newline at end of file
+main();
diff --git a/build/azure-pipelines/product-build.yml b/build/azure-pipelines/product-build.yml
index ecf47fa1cdd59..7d4246f4b3f3d 100644
--- a/build/azure-pipelines/product-build.yml
+++ b/build/azure-pipelines/product-build.yml
@@ -1,150 +1,159 @@
+trigger: none
+pr: none
+
+schedules:
+- cron: "0 5 * * Mon-Fri"
+ displayName: Mon-Fri at 7:00
+ branches:
+ include:
+ - master
+
resources:
containers:
- container: vscode-x64
image: vscodehub.azurecr.io/vscode-linux-build-agent:x64
endpoint: VSCodeHub
+ - container: vscode-arm64
+ image: vscodehub.azurecr.io/vscode-linux-build-agent:stretch-arm64
+ endpoint: VSCodeHub
+ - container: vscode-armhf
+ image: vscodehub.azurecr.io/vscode-linux-build-agent:stretch-armhf
+ endpoint: VSCodeHub
- container: snapcraft
image: snapcore/snapcraft:stable
-jobs:
-- job: Compile
- pool:
- vmImage: 'Ubuntu-16.04'
- container: vscode-x64
- steps:
- - template: product-compile.yml
+stages:
+- stage: Compile
+ jobs:
+ - job: Compile
+ pool:
+ vmImage: 'Ubuntu-16.04'
+ container: vscode-x64
+ steps:
+ - template: product-compile.yml
-- job: Windows
- condition: and(succeeded(), eq(variables['VSCODE_COMPILE_ONLY'], 'false'), eq(variables['VSCODE_BUILD_WIN32'], 'true'))
- pool:
- vmImage: VS2017-Win2016
- variables:
- VSCODE_ARCH: x64
+- stage: Windows
dependsOn:
- Compile
- steps:
- - template: win32/product-build-win32.yml
-
-- job: Windows32
- condition: and(succeeded(), eq(variables['VSCODE_COMPILE_ONLY'], 'false'), eq(variables['VSCODE_BUILD_WIN32_32BIT'], 'true'))
+ condition: and(succeeded(), eq(variables['VSCODE_COMPILE_ONLY'], 'false'))
pool:
vmImage: VS2017-Win2016
- variables:
- VSCODE_ARCH: ia32
- dependsOn:
- - Compile
- steps:
- - template: win32/product-build-win32.yml
+ jobs:
+ - job: Windows
+ condition: and(succeeded(), eq(variables['VSCODE_BUILD_WIN32'], 'true'))
+ variables:
+ VSCODE_ARCH: x64
+ steps:
+ - template: win32/product-build-win32.yml
-- job: Linux
- condition: and(succeeded(), eq(variables['VSCODE_COMPILE_ONLY'], 'false'), eq(variables['VSCODE_BUILD_LINUX'], 'true'))
- pool:
- vmImage: 'Ubuntu-16.04'
- container: vscode-x64
- dependsOn:
- - Compile
- steps:
- - template: linux/product-build-linux.yml
+ - job: Windows32
+ condition: and(succeeded(), eq(variables['VSCODE_BUILD_WIN32_32BIT'], 'true'))
+ variables:
+ VSCODE_ARCH: ia32
+ steps:
+ - template: win32/product-build-win32.yml
-- job: LinuxSnap
- condition: and(succeeded(), eq(variables['VSCODE_COMPILE_ONLY'], 'false'), eq(variables['VSCODE_BUILD_LINUX'], 'true'))
- pool:
- vmImage: 'Ubuntu-16.04'
- container: snapcraft
- dependsOn: Linux
- steps:
- - template: linux/snap-build-linux.yml
+ - job: WindowsARM64
+ condition: and(succeeded(), eq(variables['VSCODE_BUILD_WIN32_ARM64'], 'true'))
+ variables:
+ VSCODE_ARCH: arm64
+ steps:
+ - template: win32/product-build-win32-arm64.yml
-- job: LinuxArmhf
- condition: and(succeeded(), eq(variables['VSCODE_COMPILE_ONLY'], 'false'), eq(variables['VSCODE_BUILD_LINUX_ARMHF'], 'true'))
- pool:
- vmImage: 'Ubuntu-16.04'
- variables:
- VSCODE_ARCH: armhf
+- stage: Linux
dependsOn:
- Compile
- steps:
- - template: linux/product-build-linux-multiarch.yml
-
-- job: LinuxArm64
- condition: and(succeeded(), eq(variables['VSCODE_COMPILE_ONLY'], 'false'), eq(variables['VSCODE_BUILD_LINUX_ARM64'], 'true'), ne(variables['VSCODE_QUALITY'], 'stable'))
+ condition: and(succeeded(), eq(variables['VSCODE_COMPILE_ONLY'], 'false'))
pool:
vmImage: 'Ubuntu-16.04'
- variables:
- VSCODE_ARCH: arm64
- dependsOn:
- - Compile
- steps:
- - template: linux/product-build-linux-multiarch.yml
+ jobs:
+ - job: Linux
+ condition: and(succeeded(), eq(variables['VSCODE_BUILD_LINUX'], 'true'))
+ container: vscode-x64
+ variables:
+ VSCODE_ARCH: x64
+ NPM_ARCH: x64
+ steps:
+ - template: linux/product-build-linux.yml
-- job: LinuxAlpine
- condition: and(succeeded(), eq(variables['VSCODE_COMPILE_ONLY'], 'false'), eq(variables['VSCODE_BUILD_LINUX_ALPINE'], 'true'))
- pool:
- vmImage: 'Ubuntu-16.04'
- variables:
- VSCODE_ARCH: alpine
- dependsOn:
- - Compile
- steps:
- - template: linux/product-build-linux-multiarch.yml
+ - job: LinuxSnap
+ dependsOn:
+ - Linux
+ condition: and(succeeded(), eq(variables['VSCODE_BUILD_LINUX'], 'true'))
+ container: snapcraft
+ variables:
+ VSCODE_ARCH: x64
+ steps:
+ - template: linux/snap-build-linux.yml
-- job: LinuxWeb
- condition: and(succeeded(), eq(variables['VSCODE_COMPILE_ONLY'], 'false'), eq(variables['VSCODE_BUILD_WEB'], 'true'))
- pool:
- vmImage: 'Ubuntu-16.04'
- variables:
- VSCODE_ARCH: x64
- dependsOn:
- - Compile
- steps:
- - template: web/product-build-web.yml
+ - job: LinuxArmhf
+ condition: and(succeeded(), eq(variables['VSCODE_BUILD_LINUX_ARMHF'], 'true'))
+ container: vscode-armhf
+ variables:
+ VSCODE_ARCH: armhf
+ NPM_ARCH: armv7l
+ steps:
+ - template: linux/product-build-linux.yml
-- job: macOS
- condition: and(succeeded(), eq(variables['VSCODE_COMPILE_ONLY'], 'false'), eq(variables['VSCODE_BUILD_MACOS'], 'true'))
- pool:
- vmImage: macOS 10.13
+ - job: LinuxArm64
+ condition: and(succeeded(), eq(variables['VSCODE_BUILD_LINUX_ARM64'], 'true'))
+ container: vscode-arm64
+ variables:
+ VSCODE_ARCH: arm64
+ NPM_ARCH: arm64
+ steps:
+ - template: linux/product-build-linux.yml
+
+ - job: LinuxAlpine
+ condition: and(succeeded(), eq(variables['VSCODE_BUILD_LINUX_ALPINE'], 'true'))
+ variables:
+ VSCODE_ARCH: alpine
+ steps:
+ - template: linux/product-build-linux-multiarch.yml
+
+ - job: LinuxWeb
+ condition: and(succeeded(), eq(variables['VSCODE_BUILD_WEB'], 'true'))
+ variables:
+ VSCODE_ARCH: x64
+ steps:
+ - template: web/product-build-web.yml
+
+- stage: macOS
dependsOn:
- Compile
- steps:
- - template: darwin/product-build-darwin.yml
-
-- job: Release
- condition: and(succeeded(), eq(variables['VSCODE_COMPILE_ONLY'], 'false'), or(eq(variables['VSCODE_RELEASE'], 'true'), and(or(eq(variables['VSCODE_QUALITY'], 'insider'), eq(variables['VSCODE_QUALITY'], 'exploration')), eq(variables['Build.Reason'], 'Schedule'))))
+ condition: and(succeeded(), eq(variables['VSCODE_COMPILE_ONLY'], 'false'))
pool:
- vmImage: 'Ubuntu-16.04'
+ vmImage: macOS-latest
+ jobs:
+ - job: macOS
+ condition: and(succeeded(), eq(variables['VSCODE_BUILD_MACOS'], 'true'))
+ steps:
+ - template: darwin/product-build-darwin.yml
+
+- stage: Mooncake
dependsOn:
- Windows
- - Windows32
- Linux
- - LinuxSnap
- - LinuxArmhf
- - LinuxAlpine
- macOS
- steps:
- - template: release.yml
-
-- job: Mooncake
+ condition: and(succeededOrFailed(), eq(variables['VSCODE_COMPILE_ONLY'], 'false'))
pool:
vmImage: 'Ubuntu-16.04'
- condition: and(succeededOrFailed(), eq(variables['VSCODE_COMPILE_ONLY'], 'false'))
+ jobs:
+ - job: SyncMooncake
+ displayName: Sync Mooncake
+ steps:
+ - template: sync-mooncake.yml
+
+- stage: Publish
dependsOn:
- Windows
- - Windows32
- Linux
- - LinuxSnap
- - LinuxArmhf
- - LinuxAlpine
- - LinuxWeb
- macOS
- steps:
- - template: sync-mooncake.yml
-
-trigger: none
-pr: none
-
-schedules:
-- cron: "0 5 * * Mon-Fri"
- displayName: Mon-Fri at 7:00
- branches:
- include:
- - master
+ condition: and(succeeded(), eq(variables['VSCODE_COMPILE_ONLY'], 'false'), or(eq(variables['VSCODE_RELEASE'], 'true'), and(or(eq(variables['VSCODE_QUALITY'], 'insider'), eq(variables['VSCODE_QUALITY'], 'exploration')), eq(variables['Build.Reason'], 'Schedule'))))
+ pool:
+ vmImage: 'Ubuntu-16.04'
+ jobs:
+ - job: BuildService
+ displayName: Build Service
+ steps:
+ - template: release.yml
diff --git a/build/azure-pipelines/product-compile.yml b/build/azure-pipelines/product-compile.yml
index 87c70f4f8053f..2eff40b53d106 100644
--- a/build/azure-pipelines/product-compile.yml
+++ b/build/azure-pipelines/product-compile.yml
@@ -12,23 +12,24 @@ steps:
vstsFeed: 'npm-vscode'
platformIndependent: true
alias: 'Compilation'
+ dryRun: true
- task: NodeTool@0
inputs:
- versionSpec: "12.13.0"
- condition: and(succeeded(), ne(variables['CacheRestored-Compilation'], 'true'))
+ versionSpec: "12.14.1"
+ condition: and(succeeded(), ne(variables['CacheExists-Compilation'], 'true'))
- task: geeklearningio.gl-vsts-tasks-yarn.yarn-installer-task.YarnInstaller@2
inputs:
versionSpec: "1.x"
- condition: and(succeeded(), ne(variables['CacheRestored-Compilation'], 'true'))
+ condition: and(succeeded(), ne(variables['CacheExists-Compilation'], 'true'))
- task: AzureKeyVault@1
displayName: 'Azure Key Vault: Get Secrets'
inputs:
azureSubscription: 'vscode-builds-subscription'
KeyVaultName: vscode
- condition: and(succeeded(), ne(variables['CacheRestored-Compilation'], 'true'))
+ condition: and(succeeded(), ne(variables['CacheExists-Compilation'], 'true'))
- script: |
set -e
@@ -41,7 +42,7 @@ steps:
git config user.email "vscode@microsoft.com"
git config user.name "VSCode"
displayName: Prepare tooling
- condition: and(succeeded(), ne(variables['CacheRestored-Compilation'], 'true'))
+ condition: and(succeeded(), ne(variables['CacheExists-Compilation'], 'true'))
- script: |
set -e
@@ -49,33 +50,37 @@ steps:
git fetch distro
git merge $(node -p "require('./package.json').distro")
displayName: Merge distro
- condition: and(succeeded(), ne(variables['CacheRestored-Compilation'], 'true'))
+ condition: and(succeeded(), ne(variables['CacheExists-Compilation'], 'true'))
+
+- script: |
+ echo -n $VSCODE_ARCH > .build/arch
+ displayName: Prepare arch cache flag
- task: 1ESLighthouseEng.PipelineArtifactCaching.RestoreCacheV1.RestoreCache@1
inputs:
- keyfile: 'build/.cachesalt, .yarnrc, remote/.yarnrc, **/yarn.lock, !**/node_modules/**/yarn.lock, !**/.*/**/yarn.lock'
+ keyfile: '.build/arch, build/.cachesalt, .yarnrc, remote/.yarnrc, **/yarn.lock, !**/node_modules/**/yarn.lock, !**/.*/**/yarn.lock'
targetfolder: '**/node_modules, !**/node_modules/**/node_modules'
vstsFeed: 'npm-vscode'
- condition: and(succeeded(), ne(variables['CacheRestored-Compilation'], 'true'))
+ condition: and(succeeded(), ne(variables['CacheExists-Compilation'], 'true'))
- script: |
set -e
CHILD_CONCURRENCY=1 yarn --frozen-lockfile
displayName: Install dependencies
- condition: and(succeeded(), ne(variables['CacheRestored-Compilation'], 'true'), ne(variables['CacheRestored'], 'true'))
+ condition: and(succeeded(), ne(variables['CacheExists-Compilation'], 'true'), ne(variables['CacheRestored'], 'true'))
- task: 1ESLighthouseEng.PipelineArtifactCaching.SaveCacheV1.SaveCache@1
inputs:
- keyfile: 'build/.cachesalt, .yarnrc, remote/.yarnrc, **/yarn.lock, !**/node_modules/**/yarn.lock, !**/.*/**/yarn.lock'
+ keyfile: '.build/arch, build/.cachesalt, .yarnrc, remote/.yarnrc, **/yarn.lock, !**/node_modules/**/yarn.lock, !**/.*/**/yarn.lock'
targetfolder: '**/node_modules, !**/node_modules/**/node_modules'
vstsFeed: 'npm-vscode'
- condition: and(succeeded(), ne(variables['CacheRestored-Compilation'], 'true'), ne(variables['CacheRestored'], 'true'))
+ condition: and(succeeded(), ne(variables['CacheExists-Compilation'], 'true'), ne(variables['CacheRestored'], 'true'))
- script: |
set -e
yarn postinstall
displayName: Run postinstall scripts
- condition: and(succeeded(), ne(variables['CacheRestored-Compilation'], 'true'), eq(variables['CacheRestored'], 'true'))
+ condition: and(succeeded(), ne(variables['CacheExists-Compilation'], 'true'), eq(variables['CacheRestored'], 'true'))
# Mixin must run before optimize, because the CSS loader will
# inline small SVGs
@@ -83,28 +88,28 @@ steps:
set -e
node build/azure-pipelines/mixin
displayName: Mix in quality
- condition: and(succeeded(), ne(variables['CacheRestored-Compilation'], 'true'))
+ condition: and(succeeded(), ne(variables['CacheExists-Compilation'], 'true'))
- script: |
set -e
- yarn gulp hygiene --skip-tslint
- yarn gulp tslint
+ yarn gulp hygiene
yarn monaco-compile-check
- displayName: Run hygiene, tslint and monaco compile checks
- condition: and(succeeded(), ne(variables['CacheRestored-Compilation'], 'true'), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
+ yarn valid-layers-check
+ displayName: Run hygiene, monaco compile & valid layers checks
+ condition: and(succeeded(), ne(variables['CacheExists-Compilation'], 'true'), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
- script: |
set -
./build/azure-pipelines/common/extract-telemetry.sh
displayName: Extract Telemetry
- condition: and(succeeded(), ne(variables['CacheRestored-Compilation'], 'true'))
+ condition: and(succeeded(), ne(variables['CacheExists-Compilation'], 'true'))
- script: |
set -e
AZURE_WEBVIEW_STORAGE_ACCESS_KEY="$(vscode-webview-storage-key)" \
./build/azure-pipelines/common/publish-webview.sh
displayName: Publish Webview
- condition: and(succeeded(), ne(variables['CacheRestored-Compilation'], 'true'))
+ condition: and(succeeded(), ne(variables['CacheExists-Compilation'], 'true'))
- script: |
set -e
@@ -114,14 +119,14 @@ steps:
yarn gulp minify-vscode-reh
yarn gulp minify-vscode-reh-web
displayName: Compile
- condition: and(succeeded(), ne(variables['CacheRestored-Compilation'], 'true'))
+ condition: and(succeeded(), ne(variables['CacheExists-Compilation'], 'true'))
- script: |
set -e
AZURE_STORAGE_ACCESS_KEY="$(ticino-storage-key)" \
node build/azure-pipelines/upload-sourcemaps
displayName: Upload sourcemaps
- condition: and(succeeded(), ne(variables['CacheRestored-Compilation'], 'true'))
+ condition: and(succeeded(), ne(variables['CacheExists-Compilation'], 'true'))
- script: |
set -e
@@ -129,7 +134,7 @@ steps:
AZURE_DOCUMENTDB_MASTERKEY="$(builds-docdb-key-readwrite)" \
node build/azure-pipelines/common/createBuild.js $VERSION
displayName: Create build
- condition: and(succeeded(), ne(variables['CacheRestored-Compilation'], 'true'))
+ condition: and(succeeded(), ne(variables['CacheExists-Compilation'], 'true'))
- task: 1ESLighthouseEng.PipelineArtifactCaching.SaveCacheV1.SaveCache@1
inputs:
@@ -138,4 +143,4 @@ steps:
vstsFeed: 'npm-vscode'
platformIndependent: true
alias: 'Compilation'
- condition: and(succeeded(), ne(variables['CacheRestored-Compilation'], 'true'))
+ condition: and(succeeded(), ne(variables['CacheExists-Compilation'], 'true'))
diff --git a/build/azure-pipelines/publish-types/publish-types.yml b/build/azure-pipelines/publish-types/publish-types.yml
index 1d4ab83e1ac0a..10b6aa4e16af5 100644
--- a/build/azure-pipelines/publish-types/publish-types.yml
+++ b/build/azure-pipelines/publish-types/publish-types.yml
@@ -9,7 +9,7 @@ pr: none
steps:
- task: NodeTool@0
inputs:
- versionSpec: "12.13.0"
+ versionSpec: "12.14.1"
- task: geeklearningio.gl-vsts-tasks-yarn.yarn-installer-task.YarnInstaller@2
inputs:
@@ -63,7 +63,7 @@ steps:
MESSAGE="DefinitelyTyped/DefinitelyTyped#vscode-types-$TAG_VERSION created. Endgame master, please open this link, examine changes and create a PR:"
LINK="https://github.com/DefinitelyTyped/DefinitelyTyped/compare/vscode-types-$TAG_VERSION?quick_pull=1&body=Updating%20VS%20Code%20Extension%20API.%20See%20https%3A%2F%2Fgithub.com%2Fmicrosoft%2Fvscode%2Fissues%2F70175%20for%20details."
- MESSAGE2="[@octref, @jrieken, @kmaetzel, @egamma]. Please review and merge PR to publish @types/vscode."
+ MESSAGE2="[@eamodio, @jrieken, @kmaetzel, @egamma]. Please review and merge PR to publish @types/vscode."
curl -X POST -H "Authorization: Bearer $(SLACK_TOKEN)" \
-H 'Content-type: application/json; charset=utf-8' \
diff --git a/build/azure-pipelines/publish-types/update-types.ts b/build/azure-pipelines/publish-types/update-types.ts
index a5ef449b77b8c..9603726bebfcc 100644
--- a/build/azure-pipelines/publish-types/update-types.ts
+++ b/build/azure-pipelines/publish-types/update-types.ts
@@ -36,6 +36,18 @@ function updateDTSFile(outPath: string, tag: string) {
fs.writeFileSync(outPath, newContent);
}
+function repeat(str: string, times: number): string {
+ const result = new Array(times);
+ for (let i = 0; i < times; i++) {
+ result[i] = str;
+ }
+ return result.join('');
+}
+
+function convertTabsToSpaces(str: string): string {
+ return str.replace(/\t/gm, value => repeat(' ', value.length));
+}
+
function getNewFileContent(content: string, tag: string) {
const oldheader = [
`/*---------------------------------------------------------------------------------------------`,
@@ -44,7 +56,7 @@ function getNewFileContent(content: string, tag: string) {
` *--------------------------------------------------------------------------------------------*/`
].join('\n');
- return getNewFileHeader(tag) + content.slice(oldheader.length);
+ return convertTabsToSpaces(getNewFileHeader(tag) + content.slice(oldheader.length));
}
function getNewFileHeader(tag: string) {
diff --git a/build/azure-pipelines/sync-mooncake.yml b/build/azure-pipelines/sync-mooncake.yml
index 2641830a4130f..49dfc9ced80d7 100644
--- a/build/azure-pipelines/sync-mooncake.yml
+++ b/build/azure-pipelines/sync-mooncake.yml
@@ -1,7 +1,7 @@
steps:
- task: NodeTool@0
inputs:
- versionSpec: "12.13.0"
+ versionSpec: "12.14.1"
- task: geeklearningio.gl-vsts-tasks-yarn.yarn-installer-task.YarnInstaller@2
inputs:
diff --git a/build/azure-pipelines/web/product-build-web.yml b/build/azure-pipelines/web/product-build-web.yml
index 0c338203b4d6b..7f4907aa2d9f4 100644
--- a/build/azure-pipelines/web/product-build-web.yml
+++ b/build/azure-pipelines/web/product-build-web.yml
@@ -21,7 +21,7 @@ steps:
- task: NodeTool@0
inputs:
- versionSpec: "12.13.0"
+ versionSpec: "12.14.1"
- task: geeklearningio.gl-vsts-tasks-yarn.yarn-installer-task.YarnInstaller@2
inputs:
diff --git a/build/azure-pipelines/win32/ESRPClient/packages.config b/build/azure-pipelines/win32/ESRPClient/packages.config
index d7a6f144f4778..c10bed141215a 100644
--- a/build/azure-pipelines/win32/ESRPClient/packages.config
+++ b/build/azure-pipelines/win32/ESRPClient/packages.config
@@ -1,4 +1,4 @@
-
+
diff --git a/build/azure-pipelines/win32/continuous-build-win32.yml b/build/azure-pipelines/win32/continuous-build-win32.yml
index 7e16a00f0d898..8600377139c2d 100644
--- a/build/azure-pipelines/win32/continuous-build-win32.yml
+++ b/build/azure-pipelines/win32/continuous-build-win32.yml
@@ -1,54 +1,77 @@
steps:
- task: NodeTool@0
inputs:
- versionSpec: "12.13.0"
+ versionSpec: "12.14.1"
+
- task: geeklearningio.gl-vsts-tasks-yarn.yarn-installer-task.YarnInstaller@2
inputs:
versionSpec: "1.x"
+
- task: UsePythonVersion@0
inputs:
versionSpec: '2.x'
addToPath: true
+
- task: 1ESLighthouseEng.PipelineArtifactCaching.RestoreCacheV1.RestoreCache@1
inputs:
keyfile: '.yarnrc, remote/.yarnrc, **/yarn.lock, !**/node_modules/**/yarn.lock, !**/.*/**/yarn.lock'
targetfolder: '**/node_modules, !**/node_modules/**/node_modules'
- vstsFeed: '$(ArtifactFeed)'
+ vstsFeed: 'vscode-build-cache'
+
- powershell: |
yarn --frozen-lockfile
env:
CHILD_CONCURRENCY: "1"
displayName: Install Dependencies
condition: and(succeeded(), ne(variables['CacheRestored'], 'true'))
+
- task: 1ESLighthouseEng.PipelineArtifactCaching.SaveCacheV1.SaveCache@1
inputs:
keyfile: '.yarnrc, remote/.yarnrc, **/yarn.lock, !**/node_modules/**/yarn.lock, !**/.*/**/yarn.lock'
targetfolder: '**/node_modules, !**/node_modules/**/node_modules'
- vstsFeed: '$(ArtifactFeed)'
+ vstsFeed: 'vscode-build-cache'
condition: and(succeeded(), ne(variables['CacheRestored'], 'true'))
+
- powershell: |
yarn electron
-- script: |
- yarn gulp hygiene --skip-tslint
- displayName: Run Hygiene Checks
-- script: |
- yarn gulp tslint
- displayName: Run TSLint Checks
+ displayName: Download Electron
+
- powershell: |
yarn monaco-compile-check
displayName: Run Monaco Editor Checks
+
+- script: |
+ yarn valid-layers-check
+ displayName: Run Valid Layers Checks
+
- powershell: |
yarn compile
displayName: Compile Sources
+
- powershell: |
yarn download-builtin-extensions
displayName: Download Built-in Extensions
+
- powershell: |
.\scripts\test.bat --tfs "Unit Tests"
- displayName: Run Unit Tests
+ displayName: Run Unit Tests (Electron)
+
+- powershell: |
+ yarn test-browser --browser chromium --browser firefox --tfs "Browser Unit Tests"
+ displayName: Run Unit Tests (Browser)
+
- powershell: |
.\scripts\test-integration.bat --tfs "Integration Tests"
- displayName: Run Integration Tests
+ displayName: Run Integration Tests (Electron)
+
+- task: PublishPipelineArtifact@0
+ displayName: 'Publish Crash Reports'
+ inputs:
+ artifactName: crash-dump-windows
+ targetPath: .build\crashes
+ continueOnError: true
+ condition: failed()
+
- task: PublishTestResults@2
displayName: Publish Tests Results
inputs:
diff --git a/build/azure-pipelines/win32/product-build-win32-arm64.yml b/build/azure-pipelines/win32/product-build-win32-arm64.yml
new file mode 100644
index 0000000000000..ecb50ad678ed2
--- /dev/null
+++ b/build/azure-pipelines/win32/product-build-win32-arm64.yml
@@ -0,0 +1,190 @@
+steps:
+- powershell: |
+ mkdir .build -ea 0
+ "$env:BUILD_SOURCEVERSION" | Out-File -Encoding ascii -NoNewLine .build\commit
+ "$env:VSCODE_QUALITY" | Out-File -Encoding ascii -NoNewLine .build\quality
+ displayName: Prepare cache flag
+
+- task: 1ESLighthouseEng.PipelineArtifactCaching.RestoreCacheV1.RestoreCache@1
+ inputs:
+ keyfile: 'build/.cachesalt, .build/commit, .build/quality'
+ targetfolder: '.build, out-build, out-vscode-min, out-vscode-reh-min, out-vscode-reh-web-min'
+ vstsFeed: 'npm-vscode'
+ platformIndependent: true
+ alias: 'Compilation'
+
+- powershell: |
+ $ErrorActionPreference = "Stop"
+ exit 1
+ displayName: Check RestoreCache
+ condition: and(succeeded(), ne(variables['CacheRestored-Compilation'], 'true'))
+
+- task: NodeTool@0
+ inputs:
+ versionSpec: "12.14.1"
+
+- task: geeklearningio.gl-vsts-tasks-yarn.yarn-installer-task.YarnInstaller@2
+ inputs:
+ versionSpec: "1.x"
+
+- task: UsePythonVersion@0
+ inputs:
+ versionSpec: '2.x'
+ addToPath: true
+
+- task: AzureKeyVault@1
+ displayName: 'Azure Key Vault: Get Secrets'
+ inputs:
+ azureSubscription: 'vscode-builds-subscription'
+ KeyVaultName: vscode
+
+- powershell: |
+ . build/azure-pipelines/win32/exec.ps1
+ $ErrorActionPreference = "Stop"
+ "machine github.com`nlogin vscode`npassword $(github-distro-mixin-password)" | Out-File "$env:USERPROFILE\_netrc" -Encoding ASCII
+
+ exec { git config user.email "vscode@microsoft.com" }
+ exec { git config user.name "VSCode" }
+
+ mkdir .build -ea 0
+ "$(VSCODE_ARCH)" | Out-File -Encoding ascii -NoNewLine .build\arch
+ displayName: Prepare tooling
+
+- powershell: |
+ . build/azure-pipelines/win32/exec.ps1
+ $ErrorActionPreference = "Stop"
+ exec { git remote add distro "https://github.com/$(VSCODE_MIXIN_REPO).git" }
+ exec { git fetch distro }
+ exec { git merge $(node -p "require('./package.json').distro") }
+ displayName: Merge distro
+
+- task: 1ESLighthouseEng.PipelineArtifactCaching.RestoreCacheV1.RestoreCache@1
+ inputs:
+ keyfile: 'build/.cachesalt, .build/arch, .yarnrc, remote/.yarnrc, **/yarn.lock, !**/node_modules/**/yarn.lock, !**/.*/**/yarn.lock'
+ targetfolder: '**/node_modules, !**/node_modules/**/node_modules'
+ vstsFeed: 'npm-vscode'
+
+- powershell: |
+ . build/azure-pipelines/win32/exec.ps1
+ $ErrorActionPreference = "Stop"
+ $env:npm_config_arch="$(VSCODE_ARCH)"
+ $env:CHILD_CONCURRENCY="1"
+ exec { yarn --frozen-lockfile }
+ displayName: Install dependencies
+ condition: and(succeeded(), ne(variables['CacheRestored'], 'true'))
+
+- task: 1ESLighthouseEng.PipelineArtifactCaching.SaveCacheV1.SaveCache@1
+ inputs:
+ keyfile: 'build/.cachesalt, .build/arch, .yarnrc, remote/.yarnrc, **/yarn.lock, !**/node_modules/**/yarn.lock, !**/.*/**/yarn.lock'
+ targetfolder: '**/node_modules, !**/node_modules/**/node_modules'
+ vstsFeed: 'npm-vscode'
+ condition: and(succeeded(), ne(variables['CacheRestored'], 'true'))
+
+- powershell: |
+ . build/azure-pipelines/win32/exec.ps1
+ $ErrorActionPreference = "Stop"
+ exec { yarn postinstall }
+ displayName: Run postinstall scripts
+ condition: and(succeeded(), eq(variables['CacheRestored'], 'true'))
+
+- powershell: |
+ . build/azure-pipelines/win32/exec.ps1
+ $ErrorActionPreference = "Stop"
+ exec { node build/azure-pipelines/mixin }
+ displayName: Mix in quality
+
+- powershell: |
+ . build/azure-pipelines/win32/exec.ps1
+ $ErrorActionPreference = "Stop"
+ $env:VSCODE_MIXIN_PASSWORD="$(github-distro-mixin-password)"
+ exec { yarn gulp "vscode-win32-$env:VSCODE_ARCH-min-ci" }
+ exec { yarn gulp "vscode-win32-$env:VSCODE_ARCH-code-helper" }
+ exec { yarn gulp "vscode-win32-$env:VSCODE_ARCH-inno-updater" }
+ displayName: Build
+
+- task: SFP.build-tasks.custom-build-task-1.EsrpCodeSigning@1
+ inputs:
+ ConnectedServiceName: 'ESRP CodeSign'
+ FolderPath: '$(agent.builddirectory)/VSCode-win32-$(VSCODE_ARCH)'
+ Pattern: '*.dll,*.exe,*.node'
+ signConfigType: inlineSignParams
+ inlineOperation: |
+ [
+ {
+ "keyCode": "CP-230012",
+ "operationSetCode": "SigntoolSign",
+ "parameters": [
+ {
+ "parameterName": "OpusName",
+ "parameterValue": "VS Code"
+ },
+ {
+ "parameterName": "OpusInfo",
+ "parameterValue": "https://code.visualstudio.com/"
+ },
+ {
+ "parameterName": "Append",
+ "parameterValue": "/as"
+ },
+ {
+ "parameterName": "FileDigest",
+ "parameterValue": "/fd \"SHA256\""
+ },
+ {
+ "parameterName": "PageHash",
+ "parameterValue": "/NPH"
+ },
+ {
+ "parameterName": "TimeStamp",
+ "parameterValue": "/tr \"http://rfc3161.gtm.corp.microsoft.com/TSS/HttpTspServer\" /td sha256"
+ }
+ ],
+ "toolName": "sign",
+ "toolVersion": "1.0"
+ },
+ {
+ "keyCode": "CP-230012",
+ "operationSetCode": "SigntoolVerify",
+ "parameters": [
+ {
+ "parameterName": "VerifyAll",
+ "parameterValue": "/all"
+ }
+ ],
+ "toolName": "sign",
+ "toolVersion": "1.0"
+ }
+ ]
+ SessionTimeout: 120
+
+- task: NuGetCommand@2
+ displayName: Install ESRPClient.exe
+ inputs:
+ restoreSolution: 'build\azure-pipelines\win32\ESRPClient\packages.config'
+ feedsToUse: config
+ nugetConfigPath: 'build\azure-pipelines\win32\ESRPClient\NuGet.config'
+ externalFeedCredentials: 3fc0b7f7-da09-4ae7-a9c8-d69824b1819b
+ restoreDirectory: packages
+
+- task: ESRPImportCertTask@1
+ displayName: Import ESRP Request Signing Certificate
+ inputs:
+ ESRP: 'ESRP CodeSign'
+
+- powershell: |
+ $ErrorActionPreference = "Stop"
+ .\build\azure-pipelines\win32\import-esrp-auth-cert.ps1 -AuthCertificateBase64 $(esrp-auth-certificate) -AuthCertificateKey $(esrp-auth-certificate-key)
+ displayName: Import ESRP Auth Certificate
+
+- powershell: |
+ . build/azure-pipelines/win32/exec.ps1
+ $ErrorActionPreference = "Stop"
+ $env:AZURE_STORAGE_ACCESS_KEY_2 = "$(vscode-storage-key)"
+ $env:AZURE_DOCUMENTDB_MASTERKEY = "$(builds-docdb-key-readwrite)"
+ $env:VSCODE_MIXIN_PASSWORD="$(github-distro-mixin-password)"
+ .\build\azure-pipelines\win32\publish.ps1
+ displayName: Publish
+
+- task: ms.vss-governance-buildtask.governance-build-task-component-detection.ComponentGovernanceComponentDetection@0
+ displayName: 'Component Detection'
+ continueOnError: true
diff --git a/build/azure-pipelines/win32/product-build-win32.yml b/build/azure-pipelines/win32/product-build-win32.yml
index 4c9336c6c1c5a..be80731a7ab2f 100644
--- a/build/azure-pipelines/win32/product-build-win32.yml
+++ b/build/azure-pipelines/win32/product-build-win32.yml
@@ -21,7 +21,7 @@ steps:
- task: NodeTool@0
inputs:
- versionSpec: "12.13.0"
+ versionSpec: "12.14.1"
- task: geeklearningio.gl-vsts-tasks-yarn.yarn-installer-task.YarnInstaller@2
inputs:
@@ -109,7 +109,14 @@ steps:
$ErrorActionPreference = "Stop"
exec { yarn electron $(VSCODE_ARCH) }
exec { .\scripts\test.bat --build --tfs "Unit Tests" }
- displayName: Run unit tests
+ displayName: Run unit tests (Electron)
+ condition: and(succeeded(), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
+
+- powershell: |
+ . build/azure-pipelines/win32/exec.ps1
+ $ErrorActionPreference = "Stop"
+ exec { yarn test-browser --build --browser chromium --browser firefox --tfs "Browser Unit Tests" }
+ displayName: Run unit tests (Browser)
condition: and(succeeded(), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
- powershell: |
@@ -122,9 +129,41 @@ steps:
$AppProductJson = Get-Content -Raw -Path "$AppRoot\resources\app\product.json" | ConvertFrom-Json
$AppNameShort = $AppProductJson.nameShort
exec { $env:INTEGRATION_TEST_ELECTRON_PATH = "$AppRoot\$AppNameShort.exe"; $env:VSCODE_REMOTE_SERVER_PATH = "$(agent.builddirectory)\vscode-reh-win32-$(VSCODE_ARCH)"; .\scripts\test-integration.bat --build --tfs "Integration Tests" }
- displayName: Run integration tests
+ displayName: Run integration tests (Electron)
+ condition: and(succeeded(), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
+
+- powershell: |
+ . build/azure-pipelines/win32/exec.ps1
+ $ErrorActionPreference = "Stop"
+ exec { $env:VSCODE_REMOTE_SERVER_PATH = "$(agent.builddirectory)\vscode-reh-web-win32-$(VSCODE_ARCH)"; .\resources\server\test\test-web-integration.bat --browser firefox }
+ displayName: Run integration tests (Browser)
condition: and(succeeded(), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
+- powershell: |
+ . build/azure-pipelines/win32/exec.ps1
+ $ErrorActionPreference = "Stop"
+ $AppRoot = "$(agent.builddirectory)\VSCode-win32-$(VSCODE_ARCH)"
+ $AppProductJson = Get-Content -Raw -Path "$AppRoot\resources\app\product.json" | ConvertFrom-Json
+ $AppNameShort = $AppProductJson.nameShort
+ exec { $env:INTEGRATION_TEST_ELECTRON_PATH = "$AppRoot\$AppNameShort.exe"; $env:VSCODE_REMOTE_SERVER_PATH = "$(agent.builddirectory)\vscode-reh-win32-$(VSCODE_ARCH)"; .\resources\server\test\test-remote-integration.bat }
+ displayName: Run remote integration tests (Electron)
+ condition: and(succeeded(), eq(variables['VSCODE_STEP_ON_IT'], 'false'))
+
+- task: PublishPipelineArtifact@0
+ inputs:
+ artifactName: crash-dump-windows-$(VSCODE_ARCH)
+ targetPath: .build\crashes
+ displayName: 'Publish Crash Reports'
+ continueOnError: true
+ condition: failed()
+
+- task: PublishTestResults@2
+ displayName: Publish Tests Results
+ inputs:
+ testResultsFiles: '*-results.xml'
+ searchFolder: '$(Build.ArtifactStagingDirectory)/test-results'
+ condition: succeededOrFailed()
+
- task: SFP.build-tasks.custom-build-task-1.EsrpCodeSigning@1
inputs:
ConnectedServiceName: 'ESRP CodeSign'
@@ -186,7 +225,7 @@ steps:
restoreSolution: 'build\azure-pipelines\win32\ESRPClient\packages.config'
feedsToUse: config
nugetConfigPath: 'build\azure-pipelines\win32\ESRPClient\NuGet.config'
- externalFeedCredentials: 3fc0b7f7-da09-4ae7-a9c8-d69824b1819b
+ externalFeedCredentials: 'ESRP Nuget'
restoreDirectory: packages
- task: ESRPImportCertTask@1
@@ -204,7 +243,6 @@ steps:
$ErrorActionPreference = "Stop"
$env:AZURE_STORAGE_ACCESS_KEY_2 = "$(vscode-storage-key)"
$env:AZURE_DOCUMENTDB_MASTERKEY = "$(builds-docdb-key-readwrite)"
- $env:VSCODE_HOCKEYAPP_TOKEN = "$(vscode-hockeyapp-token)"
$env:VSCODE_MIXIN_PASSWORD="$(github-distro-mixin-password)"
.\build\azure-pipelines\win32\publish.ps1
displayName: Publish
diff --git a/build/azure-pipelines/win32/publish.ps1 b/build/azure-pipelines/win32/publish.ps1
index 5a22d4749cf6c..a225f9d5fdf9c 100644
--- a/build/azure-pipelines/win32/publish.ps1
+++ b/build/azure-pipelines/win32/publish.ps1
@@ -11,26 +11,26 @@ $SystemExe = "$Repo\.build\win32-$Arch\system-setup\VSCodeSetup.exe"
$UserExe = "$Repo\.build\win32-$Arch\user-setup\VSCodeSetup.exe"
$Zip = "$Repo\.build\win32-$Arch\archive\VSCode-win32-$Arch.zip"
$LegacyServer = "$Root\vscode-reh-win32-$Arch"
-$ServerName = "vscode-server-win32-$Arch"
-$Server = "$Root\$ServerName"
+$Server = "$Root\vscode-server-win32-$Arch"
$ServerZip = "$Repo\.build\vscode-server-win32-$Arch.zip"
$Build = "$Root\VSCode-win32-$Arch"
# Create server archive
-exec { Rename-Item -Path $LegacyServer -NewName $ServerName }
-exec { .\node_modules\7zip\7zip-lite\7z.exe a -tzip $ServerZip $Server -r }
+if ("$Arch" -ne "arm64") {
+ exec { xcopy $LegacyServer $Server /H /E /I }
+ exec { .\node_modules\7zip\7zip-lite\7z.exe a -tzip $ServerZip $Server -r }
+}
# get version
$PackageJson = Get-Content -Raw -Path "$Build\resources\app\package.json" | ConvertFrom-Json
$Version = $PackageJson.version
-$AssetPlatform = if ("$Arch" -eq "ia32") { "win32" } else { "win32-x64" }
+$AssetPlatform = if ("$Arch" -eq "ia32") { "win32" } else { "win32-$Arch" }
exec { node build/azure-pipelines/common/createAsset.js "$AssetPlatform-archive" archive "VSCode-win32-$Arch-$Version.zip" $Zip }
exec { node build/azure-pipelines/common/createAsset.js "$AssetPlatform" setup "VSCodeSetup-$Arch-$Version.exe" $SystemExe }
exec { node build/azure-pipelines/common/createAsset.js "$AssetPlatform-user" setup "VSCodeUserSetup-$Arch-$Version.exe" $UserExe }
-exec { node build/azure-pipelines/common/createAsset.js "server-$AssetPlatform" archive "vscode-server-win32-$Arch.zip" $ServerZip }
-# publish hockeyapp symbols
-$hockeyAppId = if ("$Arch" -eq "ia32") { "$env:VSCODE_HOCKEYAPP_ID_WIN32" } else { "$env:VSCODE_HOCKEYAPP_ID_WIN64" }
-exec { node build/azure-pipelines/common/symbols.js "$env:VSCODE_MIXIN_PASSWORD" "$env:VSCODE_HOCKEYAPP_TOKEN" "$Arch" $hockeyAppId }
+if ("$Arch" -ne "arm64") {
+ exec { node build/azure-pipelines/common/createAsset.js "server-$AssetPlatform" archive "vscode-server-win32-$Arch.zip" $ServerZip }
+}
diff --git a/build/azure-pipelines/win32/sign.ps1 b/build/azure-pipelines/win32/sign.ps1
index 00c4d42d9dbff..840cbe4071f8a 100644
--- a/build/azure-pipelines/win32/sign.ps1
+++ b/build/azure-pipelines/win32/sign.ps1
@@ -67,4 +67,4 @@ $Input = Create-TmpJson @{
$Output = [System.IO.Path]::GetTempFileName()
$ScriptPath = Split-Path -Path $MyInvocation.MyCommand.Definition -Parent
-& "$ScriptPath\ESRPClient\packages\EsrpClient.1.0.27\tools\ESRPClient.exe" Sign -a $Auth -p $Policy -i $Input -o $Output
\ No newline at end of file
+& "$ScriptPath\ESRPClient\packages\Microsoft.ESRPClient.1.2.25\tools\ESRPClient.exe" Sign -a $Auth -p $Policy -i $Input -o $Output
diff --git a/build/builtInExtensions.json b/build/builtInExtensions.json
deleted file mode 100644
index 15fc5ec1d2952..0000000000000
--- a/build/builtInExtensions.json
+++ /dev/null
@@ -1,47 +0,0 @@
-[
- {
- "name": "ms-vscode.node-debug",
- "version": "1.40.1",
- "repo": "https://github.com/Microsoft/vscode-node-debug",
- "metadata": {
- "id": "b6ded8fb-a0a0-4c1c-acbd-ab2a3bc995a6",
- "publisherId": {
- "publisherId": "5f5636e7-69ed-4afe-b5d6-8d231fb3d3ee",
- "publisherName": "ms-vscode",
- "displayName": "Microsoft",
- "flags": "verified"
- },
- "publisherDisplayName": "Microsoft"
- }
- },
- {
- "name": "ms-vscode.node-debug2",
- "version": "1.39.3",
- "repo": "https://github.com/Microsoft/vscode-node-debug2",
- "metadata": {
- "id": "36d19e17-7569-4841-a001-947eb18602b2",
- "publisherId": {
- "publisherId": "5f5636e7-69ed-4afe-b5d6-8d231fb3d3ee",
- "publisherName": "ms-vscode",
- "displayName": "Microsoft",
- "flags": "verified"
- },
- "publisherDisplayName": "Microsoft"
- }
- },
- {
- "name": "ms-vscode.references-view",
- "version": "0.0.31",
- "repo": "https://github.com/Microsoft/vscode-reference-view",
- "metadata": {
- "id": "dc489f46-520d-4556-ae85-1f9eab3c412d",
- "publisherId": {
- "publisherId": "5f5636e7-69ed-4afe-b5d6-8d231fb3d3ee",
- "publisherName": "ms-vscode",
- "displayName": "Microsoft",
- "flags": "verified"
- },
- "publisherDisplayName": "Microsoft"
- }
- }
-]
diff --git a/build/builtin/browser-main.js b/build/builtin/browser-main.js
index 60b30655c0cb3..e5956179567c9 100644
--- a/build/builtin/browser-main.js
+++ b/build/builtin/browser-main.js
@@ -6,11 +6,10 @@
const fs = require('fs');
const path = require('path');
const os = require('os');
-// @ts-ignore review
const { remote } = require('electron');
const dialog = remote.dialog;
-const builtInExtensionsPath = path.join(__dirname, '..', 'builtInExtensions.json');
+const builtInExtensionsPath = path.join(__dirname, '..', '..', 'product.json');
const controlFilePath = path.join(os.homedir(), '.vscode-oss-dev', 'extensions', 'control.json');
function readJson(filePath) {
@@ -111,7 +110,7 @@ function render(el, state) {
function main() {
const el = document.getElementById('extensions');
- const builtin = readJson(builtInExtensionsPath);
+ const builtin = readJson(builtInExtensionsPath).builtInExtensions;
let control;
try {
@@ -123,4 +122,4 @@ function main() {
render(el, { builtin, control });
}
-window.onload = main;
\ No newline at end of file
+window.onload = main;
diff --git a/build/builtin/main.js b/build/builtin/main.js
index b094a67cac5c7..7379de7a93d2e 100644
--- a/build/builtin/main.js
+++ b/build/builtin/main.js
@@ -10,11 +10,11 @@ const path = require('path');
let window = null;
app.once('ready', () => {
- window = new BrowserWindow({ width: 800, height: 600, webPreferences: { nodeIntegration: true, webviewTag: true } });
+ window = new BrowserWindow({ width: 800, height: 600, webPreferences: { nodeIntegration: true, webviewTag: true, enableWebSQL: false, nativeWindowOpen: true } });
window.setMenuBarVisibility(false);
window.loadURL(url.format({ pathname: path.join(__dirname, 'index.html'), protocol: 'file:', slashes: true }));
// window.webContents.openDevTools();
window.once('closed', () => window = null);
});
-app.on('window-all-closed', () => app.quit());
\ No newline at end of file
+app.on('window-all-closed', () => app.quit());
diff --git a/build/darwin/sign.js b/build/darwin/sign.js
new file mode 100644
index 0000000000000..b8eb9fc752503
--- /dev/null
+++ b/build/darwin/sign.js
@@ -0,0 +1,61 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+'use strict';
+Object.defineProperty(exports, "__esModule", { value: true });
+const codesign = require("electron-osx-sign");
+const path = require("path");
+const util = require("../lib/util");
+const product = require("../../product.json");
+async function main() {
+ const buildDir = process.env['AGENT_BUILDDIRECTORY'];
+ const tempDir = process.env['AGENT_TEMPDIRECTORY'];
+ if (!buildDir) {
+ throw new Error('$AGENT_BUILDDIRECTORY not set');
+ }
+ if (!tempDir) {
+ throw new Error('$AGENT_TEMPDIRECTORY not set');
+ }
+ const baseDir = path.dirname(__dirname);
+ const appRoot = path.join(buildDir, 'VSCode-darwin');
+ const appName = product.nameLong + '.app';
+ const appFrameworkPath = path.join(appRoot, appName, 'Contents', 'Frameworks');
+ const helperAppBaseName = product.nameShort;
+ const gpuHelperAppName = helperAppBaseName + ' Helper (GPU).app';
+ const pluginHelperAppName = helperAppBaseName + ' Helper (Plugin).app';
+ const rendererHelperAppName = helperAppBaseName + ' Helper (Renderer).app';
+ const defaultOpts = {
+ app: path.join(appRoot, appName),
+ platform: 'darwin',
+ entitlements: path.join(baseDir, 'azure-pipelines', 'darwin', 'app-entitlements.plist'),
+ 'entitlements-inherit': path.join(baseDir, 'azure-pipelines', 'darwin', 'app-entitlements.plist'),
+ hardenedRuntime: true,
+ 'pre-auto-entitlements': false,
+ 'pre-embed-provisioning-profile': false,
+ keychain: path.join(tempDir, 'buildagent.keychain'),
+ version: util.getElectronVersion(),
+ identity: '99FM488X57',
+ 'gatekeeper-assess': false
+ };
+ const appOpts = Object.assign(Object.assign({}, defaultOpts), {
+ // TODO(deepak1556): Incorrectly declared type in electron-osx-sign
+ ignore: (filePath) => {
+ return filePath.includes(gpuHelperAppName) ||
+ filePath.includes(pluginHelperAppName) ||
+ filePath.includes(rendererHelperAppName);
+ } });
+ const gpuHelperOpts = Object.assign(Object.assign({}, defaultOpts), { app: path.join(appFrameworkPath, gpuHelperAppName), entitlements: path.join(baseDir, 'azure-pipelines', 'darwin', 'helper-gpu-entitlements.plist'), 'entitlements-inherit': path.join(baseDir, 'azure-pipelines', 'darwin', 'helper-gpu-entitlements.plist') });
+ const pluginHelperOpts = Object.assign(Object.assign({}, defaultOpts), { app: path.join(appFrameworkPath, pluginHelperAppName), entitlements: path.join(baseDir, 'azure-pipelines', 'darwin', 'helper-plugin-entitlements.plist'), 'entitlements-inherit': path.join(baseDir, 'azure-pipelines', 'darwin', 'helper-plugin-entitlements.plist') });
+ const rendererHelperOpts = Object.assign(Object.assign({}, defaultOpts), { app: path.join(appFrameworkPath, rendererHelperAppName), entitlements: path.join(baseDir, 'azure-pipelines', 'darwin', 'helper-renderer-entitlements.plist'), 'entitlements-inherit': path.join(baseDir, 'azure-pipelines', 'darwin', 'helper-renderer-entitlements.plist') });
+ await codesign.signAsync(gpuHelperOpts);
+ await codesign.signAsync(pluginHelperOpts);
+ await codesign.signAsync(rendererHelperOpts);
+ await codesign.signAsync(appOpts);
+}
+if (require.main === module) {
+ main().catch(err => {
+ console.error(err);
+ process.exit(1);
+ });
+}
diff --git a/build/darwin/sign.ts b/build/darwin/sign.ts
new file mode 100644
index 0000000000000..ee5d2eeb17b84
--- /dev/null
+++ b/build/darwin/sign.ts
@@ -0,0 +1,90 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+'use strict';
+
+import * as codesign from 'electron-osx-sign';
+import * as path from 'path';
+import * as util from '../lib/util';
+import * as product from '../../product.json';
+
+async function main(): Promise {
+ const buildDir = process.env['AGENT_BUILDDIRECTORY'];
+ const tempDir = process.env['AGENT_TEMPDIRECTORY'];
+
+ if (!buildDir) {
+ throw new Error('$AGENT_BUILDDIRECTORY not set');
+ }
+
+ if (!tempDir) {
+ throw new Error('$AGENT_TEMPDIRECTORY not set');
+ }
+
+ const baseDir = path.dirname(__dirname);
+ const appRoot = path.join(buildDir, 'VSCode-darwin');
+ const appName = product.nameLong + '.app';
+ const appFrameworkPath = path.join(appRoot, appName, 'Contents', 'Frameworks');
+ const helperAppBaseName = product.nameShort;
+ const gpuHelperAppName = helperAppBaseName + ' Helper (GPU).app';
+ const pluginHelperAppName = helperAppBaseName + ' Helper (Plugin).app';
+ const rendererHelperAppName = helperAppBaseName + ' Helper (Renderer).app';
+
+ const defaultOpts: codesign.SignOptions = {
+ app: path.join(appRoot, appName),
+ platform: 'darwin',
+ entitlements: path.join(baseDir, 'azure-pipelines', 'darwin', 'app-entitlements.plist'),
+ 'entitlements-inherit': path.join(baseDir, 'azure-pipelines', 'darwin', 'app-entitlements.plist'),
+ hardenedRuntime: true,
+ 'pre-auto-entitlements': false,
+ 'pre-embed-provisioning-profile': false,
+ keychain: path.join(tempDir, 'buildagent.keychain'),
+ version: util.getElectronVersion(),
+ identity: '99FM488X57',
+ 'gatekeeper-assess': false
+ };
+
+ const appOpts = {
+ ...defaultOpts,
+ // TODO(deepak1556): Incorrectly declared type in electron-osx-sign
+ ignore: (filePath: string) => {
+ return filePath.includes(gpuHelperAppName) ||
+ filePath.includes(pluginHelperAppName) ||
+ filePath.includes(rendererHelperAppName);
+ }
+ };
+
+ const gpuHelperOpts: codesign.SignOptions = {
+ ...defaultOpts,
+ app: path.join(appFrameworkPath, gpuHelperAppName),
+ entitlements: path.join(baseDir, 'azure-pipelines', 'darwin', 'helper-gpu-entitlements.plist'),
+ 'entitlements-inherit': path.join(baseDir, 'azure-pipelines', 'darwin', 'helper-gpu-entitlements.plist'),
+ };
+
+ const pluginHelperOpts: codesign.SignOptions = {
+ ...defaultOpts,
+ app: path.join(appFrameworkPath, pluginHelperAppName),
+ entitlements: path.join(baseDir, 'azure-pipelines', 'darwin', 'helper-plugin-entitlements.plist'),
+ 'entitlements-inherit': path.join(baseDir, 'azure-pipelines', 'darwin', 'helper-plugin-entitlements.plist'),
+ };
+
+ const rendererHelperOpts: codesign.SignOptions = {
+ ...defaultOpts,
+ app: path.join(appFrameworkPath, rendererHelperAppName),
+ entitlements: path.join(baseDir, 'azure-pipelines', 'darwin', 'helper-renderer-entitlements.plist'),
+ 'entitlements-inherit': path.join(baseDir, 'azure-pipelines', 'darwin', 'helper-renderer-entitlements.plist'),
+ };
+
+ await codesign.signAsync(gpuHelperOpts);
+ await codesign.signAsync(pluginHelperOpts);
+ await codesign.signAsync(rendererHelperOpts);
+ await codesign.signAsync(appOpts as any);
+}
+
+if (require.main === module) {
+ main().catch(err => {
+ console.error(err);
+ process.exit(1);
+ });
+}
diff --git a/build/gulpfile.editor.js b/build/gulpfile.editor.js
index 6734a820cf181..6cb65c5a16681 100644
--- a/build/gulpfile.editor.js
+++ b/build/gulpfile.editor.js
@@ -16,15 +16,17 @@ const cp = require('child_process');
const compilation = require('./lib/compilation');
const monacoapi = require('./monaco/api');
const fs = require('fs');
+const webpack = require('webpack');
+const webpackGulp = require('webpack-stream');
-var root = path.dirname(__dirname);
-var sha1 = util.getVersion(root);
-var semver = require('./monaco/package.json').version;
-var headerVersion = semver + '(' + sha1 + ')';
+let root = path.dirname(__dirname);
+let sha1 = util.getVersion(root);
+let semver = require('./monaco/package.json').version;
+let headerVersion = semver + '(' + sha1 + ')';
// Build
-var editorEntryPoints = [
+let editorEntryPoints = [
{
name: 'vs/editor/editor.main',
include: [],
@@ -40,11 +42,11 @@ var editorEntryPoints = [
}
];
-var editorResources = [
- 'out-editor-build/vs/base/browser/ui/codiconLabel/**/*.ttf'
+let editorResources = [
+ 'out-editor-build/vs/base/browser/ui/codicons/**/*.ttf'
];
-var BUNDLED_FILE_HEADER = [
+let BUNDLED_FILE_HEADER = [
'/*!-----------------------------------------------------------',
' * Copyright (c) Microsoft Corporation. All rights reserved.',
' * Version: ' + headerVersion,
@@ -57,7 +59,6 @@ var BUNDLED_FILE_HEADER = [
const languages = i18n.defaultLanguages.concat([]); // i18n.defaultLanguages.concat(process.env.VSCODE_QUALITY !== 'stable' ? i18n.extraLanguages : []);
const extractEditorSrcTask = task.define('extract-editor-src', () => {
- console.log(`If the build fails, consider tweaking shakeLevel below to a lower value.`);
const apiusages = monacoapi.execute().usageContent;
const extrausages = fs.readFileSync(path.join(root, 'build', 'monaco', 'monaco.usage.recipe')).toString();
standalone.extractEditor({
@@ -71,21 +72,8 @@ const extractEditorSrcTask = task.define('extract-editor-src', () => {
apiusages,
extrausages
],
- typings: [
- 'typings/lib.ie11_safe_es6.d.ts',
- 'typings/thenable.d.ts',
- 'typings/es6-promise.d.ts',
- 'typings/require-monaco.d.ts',
- "typings/lib.es2018.promise.d.ts",
- 'vs/monaco.d.ts'
- ],
- libs: [
- `lib.es5.d.ts`,
- `lib.dom.d.ts`,
- `lib.webworker.importscripts.d.ts`
- ],
shakeLevel: 2, // 0-Files, 1-InnerFile, 2-ClassMembers
- importIgnorePattern: /(^vs\/css!)|(promise-polyfill\/polyfill)/,
+ importIgnorePattern: /(^vs\/css!)/,
destRoot: path.join(root, 'out-editor-src'),
redirects: []
});
@@ -138,23 +126,81 @@ const createESMSourcesAndResourcesTask = task.define('extract-editor-esm', () =>
});
const compileEditorESMTask = task.define('compile-editor-esm', () => {
+ const KEEP_PREV_ANALYSIS = false;
+ const FAIL_ON_PURPOSE = false;
+ console.log(`Launching the TS compiler at ${path.join(__dirname, '../out-editor-esm')}...`);
+ let result;
if (process.platform === 'win32') {
- const result = cp.spawnSync(`..\\node_modules\\.bin\\tsc.cmd`, {
+ result = cp.spawnSync(`..\\node_modules\\.bin\\tsc.cmd`, {
cwd: path.join(__dirname, '../out-editor-esm')
});
- console.log(result.stdout.toString());
- console.log(result.stderr.toString());
} else {
- const result = cp.spawnSync(`node`, [`../node_modules/.bin/tsc`], {
+ result = cp.spawnSync(`node`, [`../node_modules/.bin/tsc`], {
cwd: path.join(__dirname, '../out-editor-esm')
});
- console.log(result.stdout.toString());
- console.log(result.stderr.toString());
+ }
+
+ console.log(result.stdout.toString());
+ console.log(result.stderr.toString());
+
+ if (FAIL_ON_PURPOSE || result.status !== 0) {
+ console.log(`The TS Compilation failed, preparing analysis folder...`);
+ const destPath = path.join(__dirname, '../../vscode-monaco-editor-esm-analysis');
+ const keepPrevAnalysis = (KEEP_PREV_ANALYSIS && fs.existsSync(destPath));
+ const cleanDestPath = (keepPrevAnalysis ? Promise.resolve() : util.rimraf(destPath)());
+ return cleanDestPath.then(() => {
+ // build a list of files to copy
+ const files = util.rreddir(path.join(__dirname, '../out-editor-esm'));
+
+ if (!keepPrevAnalysis) {
+ fs.mkdirSync(destPath);
+
+ // initialize a new repository
+ cp.spawnSync(`git`, [`init`], {
+ cwd: destPath
+ });
+
+ // copy files from src
+ for (const file of files) {
+ const srcFilePath = path.join(__dirname, '../src', file);
+ const dstFilePath = path.join(destPath, file);
+ if (fs.existsSync(srcFilePath)) {
+ util.ensureDir(path.dirname(dstFilePath));
+ const contents = fs.readFileSync(srcFilePath).toString().replace(/\r\n|\r|\n/g, '\n');
+ fs.writeFileSync(dstFilePath, contents);
+ }
+ }
+
+ // create an initial commit to diff against
+ cp.spawnSync(`git`, [`add`, `.`], {
+ cwd: destPath
+ });
+
+ // create the commit
+ cp.spawnSync(`git`, [`commit`, `-m`, `"original sources"`, `--no-gpg-sign`], {
+ cwd: destPath
+ });
+ }
+
+ // copy files from tree shaken src
+ for (const file of files) {
+ const srcFilePath = path.join(__dirname, '../out-editor-src', file);
+ const dstFilePath = path.join(destPath, file);
+ if (fs.existsSync(srcFilePath)) {
+ util.ensureDir(path.dirname(dstFilePath));
+ const contents = fs.readFileSync(srcFilePath).toString().replace(/\r\n|\r|\n/g, '\n');
+ fs.writeFileSync(dstFilePath, contents);
+ }
+ }
+
+ console.log(`Open in VS Code the folder at '${destPath}' and you can alayze the compilation error`);
+ throw new Error('Standalone Editor compilation failed. If this is the build machine, simply launch `yarn run gulp editor-distro` on your machine to further analyze the compilation problem.');
+ });
}
});
function toExternalDTS(contents) {
- let lines = contents.split('\n');
+ let lines = contents.split(/\r\n|\r|\n/);
let killNextCloseCurlyBrace = false;
for (let i = 0; i < lines.length; i++) {
let line = lines[i];
@@ -184,8 +230,13 @@ function toExternalDTS(contents) {
if (line.indexOf('declare namespace monaco.') === 0) {
lines[i] = line.replace('declare namespace monaco.', 'export namespace ');
}
+
+ if (line.indexOf('declare let MonacoEnvironment') === 0) {
+ lines[i] = `declare global {\n let MonacoEnvironment: Environment | undefined;\n}`;
+ // lines[i] = line.replace('declare namespace monaco.', 'export namespace ');
+ }
}
- return lines.join('\n');
+ return lines.join('\n').replace(/\n\n\n+/g, '\n\n');
}
function filterStream(testFunc) {
@@ -220,7 +271,7 @@ const finalEditorResourcesTask = task.define('final-editor-resources', () => {
// package.json
gulp.src('build/monaco/package.json')
.pipe(es.through(function (data) {
- var json = JSON.parse(data.contents.toString());
+ let json = JSON.parse(data.contents.toString());
json.private = false;
data.contents = Buffer.from(JSON.stringify(json, null, ' '));
this.emit('data', data);
@@ -264,10 +315,10 @@ const finalEditorResourcesTask = task.define('final-editor-resources', () => {
return;
}
- var relativePathToMap = path.relative(path.join(data.relative), path.join('min-maps', data.relative + '.map'));
+ let relativePathToMap = path.relative(path.join(data.relative), path.join('min-maps', data.relative + '.map'));
- var strContents = data.contents.toString();
- var newStr = '//# sourceMappingURL=' + relativePathToMap.replace(/\\/g, '/');
+ let strContents = data.contents.toString();
+ let newStr = '//# sourceMappingURL=' + relativePathToMap.replace(/\\/g, '/');
strContents = strContents.replace(/\/\/# sourceMappingURL=[^ ]+$/, newStr);
data.contents = Buffer.from(strContents);
@@ -284,6 +335,13 @@ const finalEditorResourcesTask = task.define('final-editor-resources', () => {
);
});
+gulp.task('extract-editor-src',
+ task.series(
+ util.rimraf('out-editor-src'),
+ extractEditorSrcTask
+ )
+);
+
gulp.task('editor-distro',
task.series(
task.parallel(
@@ -310,6 +368,56 @@ gulp.task('editor-distro',
)
);
+const bundleEditorESMTask = task.define('editor-esm-bundle-webpack', () => {
+ const result = es.through();
+
+ const webpackConfigPath = path.join(root, 'build/monaco/monaco.webpack.config.js');
+
+ const webpackConfig = {
+ ...require(webpackConfigPath),
+ ...{ mode: 'production' }
+ };
+
+ const webpackDone = (err, stats) => {
+ if (err) {
+ result.emit('error', err);
+ return;
+ }
+ const { compilation } = stats;
+ if (compilation.errors.length > 0) {
+ result.emit('error', compilation.errors.join('\n'));
+ }
+ if (compilation.warnings.length > 0) {
+ result.emit('data', compilation.warnings.join('\n'));
+ }
+ };
+
+ return webpackGulp(webpackConfig, webpack, webpackDone)
+ .pipe(gulp.dest('out-editor-esm-bundle'));
+});
+
+gulp.task('editor-esm-bundle',
+ task.series(
+ task.parallel(
+ util.rimraf('out-editor-src'),
+ util.rimraf('out-editor-esm'),
+ util.rimraf('out-monaco-editor-core'),
+ util.rimraf('out-editor-esm-bundle'),
+ ),
+ extractEditorSrcTask,
+ createESMSourcesAndResourcesTask,
+ compileEditorESMTask,
+ bundleEditorESMTask,
+ )
+);
+
+gulp.task('monacodts', task.define('monacodts', () => {
+ const result = monacoapi.execute();
+ fs.writeFileSync(result.filePath, result.content);
+ fs.writeFileSync(path.join(root, 'src/vs/editor/common/standalone/standaloneEnums.ts'), result.enums);
+ return Promise.resolve(true);
+}));
+
//#region monaco type checking
function createTscCompileTask(watch) {
@@ -348,10 +456,8 @@ function createTscCompileTask(watch) {
// e.g. src/vs/base/common/strings.ts(663,5): error TS2322: Type '1234' is not assignable to type 'string'.
let fullpath = path.join(root, match[1]);
let message = match[3];
- // @ts-ignore
reporter(fullpath + message);
} else {
- // @ts-ignore
reporter(str);
}
}
diff --git a/build/gulpfile.extensions.js b/build/gulpfile.extensions.js
index ca28c413a71ec..6ae72e4cf0631 100644
--- a/build/gulpfile.extensions.js
+++ b/build/gulpfile.extensions.js
@@ -8,9 +8,11 @@ require('events').EventEmitter.defaultMaxListeners = 100;
const gulp = require('gulp');
const path = require('path');
+const nodeUtil = require('util');
const tsb = require('gulp-tsb');
const es = require('event-stream');
const filter = require('gulp-filter');
+const webpack = require('webpack');
const util = require('./lib/util');
const task = require('./lib/task');
const watcher = require('./lib/watch');
@@ -21,6 +23,8 @@ const nlsDev = require('vscode-nls-dev');
const root = path.dirname(__dirname);
const commit = util.getVersion(root);
const plumber = require('gulp-plumber');
+const fancyLog = require('fancy-log');
+const ansiColors = require('ansi-colors');
const ext = require('./lib/extensions');
const extensionsPath = path.join(path.dirname(__dirname), 'extensions');
@@ -109,7 +113,8 @@ const tasks = compilations.map(function (tsconfigFile) {
const compileTask = task.define(`compile-extension:${name}`, task.series(cleanTask, () => {
const pipeline = createPipeline(false, true);
- const input = pipeline.tsProjectSrc();
+ const nonts = gulp.src(src, srcOpts).pipe(filter(['**', '!**/*.ts']));
+ const input = es.merge(nonts, pipeline.tsProjectSrc());
return input
.pipe(pipeline())
@@ -118,7 +123,8 @@ const tasks = compilations.map(function (tsconfigFile) {
const watchTask = task.define(`watch-extension:${name}`, task.series(cleanTask, () => {
const pipeline = createPipeline(false);
- const input = pipeline.tsProjectSrc();
+ const nonts = gulp.src(src, srcOpts).pipe(filter(['**', '!**/*.ts']));
+ const input = es.merge(nonts, pipeline.tsProjectSrc());
const watchInput = watcher(src, { ...srcOpts, ...{ readDelay: 200 } });
return watchInput
@@ -128,7 +134,8 @@ const tasks = compilations.map(function (tsconfigFile) {
const compileBuildTask = task.define(`compile-build-extension-${name}`, task.series(cleanTask, () => {
const pipeline = createPipeline(true, true);
- const input = pipeline.tsProjectSrc();
+ const nonts = gulp.src(src, srcOpts).pipe(filter(['**', '!**/*.ts']));
+ const input = es.merge(nonts, pipeline.tsProjectSrc());
return input
.pipe(pipeline())
@@ -158,9 +165,84 @@ gulp.task(compileExtensionsBuildLegacyTask);
const cleanExtensionsBuildTask = task.define('clean-extensions-build', util.rimraf('.build/extensions'));
const compileExtensionsBuildTask = task.define('compile-extensions-build', task.series(
cleanExtensionsBuildTask,
- task.define('bundle-extensions-build', () => ext.packageLocalExtensionsStream().pipe(gulp.dest('.build'))),
- task.define('bundle-marketplace-extensions-build', () => ext.packageMarketplaceExtensionsStream().pipe(gulp.dest('.build'))),
+ task.define('bundle-extensions-build', () => ext.packageLocalExtensionsStream(false).pipe(gulp.dest('.build'))),
+ task.define('bundle-marketplace-extensions-build', () => ext.packageMarketplaceExtensionsStream(false).pipe(gulp.dest('.build'))),
));
gulp.task(compileExtensionsBuildTask);
exports.compileExtensionsBuildTask = compileExtensionsBuildTask;
+
+const compileWebExtensionsTask = task.define('compile-web', () => buildWebExtensions(false));
+gulp.task(compileWebExtensionsTask);
+exports.compileWebExtensionsTask = compileWebExtensionsTask;
+
+const watchWebExtensionsTask = task.define('watch-web', () => buildWebExtensions(true));
+gulp.task(watchWebExtensionsTask);
+exports.watchWebExtensionsTask = watchWebExtensionsTask;
+
+async function buildWebExtensions(isWatch) {
+
+ const webpackConfigLocations = await nodeUtil.promisify(glob)(
+ path.join(extensionsPath, '**', 'extension-browser.webpack.config.js'),
+ { ignore: ['**/node_modules'] }
+ );
+
+ const webpackConfigs = [];
+
+ for (const webpackConfigPath of webpackConfigLocations) {
+ const configOrFnOrArray = require(webpackConfigPath);
+ function addConfig(configOrFn) {
+ if (typeof configOrFn === 'function') {
+ webpackConfigs.push(configOrFn({}, {}));
+ } else {
+ webpackConfigs.push(configOrFn);
+ }
+ }
+ addConfig(configOrFnOrArray);
+ }
+ function reporter(fullStats) {
+ if (Array.isArray(fullStats.children)) {
+ for (const stats of fullStats.children) {
+ const outputPath = stats.outputPath;
+ if (outputPath) {
+ const relativePath = path.relative(extensionsPath, outputPath).replace(/\\/g, '/');
+ const match = relativePath.match(/[^\/]+(\/server|\/client)?/);
+ fancyLog(`Finished ${ansiColors.green('packaging web extension')} ${ansiColors.cyan(match[0])} with ${stats.errors.length} errors.`);
+ }
+ if (Array.isArray(stats.errors)) {
+ stats.errors.forEach(error => {
+ fancyLog.error(error);
+ });
+ }
+ if (Array.isArray(stats.warnings)) {
+ stats.warnings.forEach(warning => {
+ fancyLog.warn(warning);
+ });
+ }
+ }
+ }
+ }
+ return new Promise((resolve, reject) => {
+ if (isWatch) {
+ webpack(webpackConfigs).watch({}, (err, stats) => {
+ if (err) {
+ reject();
+ } else {
+ reporter(stats.toJson());
+ }
+ });
+ } else {
+ webpack(webpackConfigs).run((err, stats) => {
+ if (err) {
+ fancyLog.error(err);
+ reject();
+ } else {
+ reporter(stats.toJson());
+ resolve();
+ }
+ });
+ }
+ });
+}
+
+
diff --git a/build/gulpfile.hygiene.js b/build/gulpfile.hygiene.js
index a8bb8898c90fb..4952ee1c26d58 100644
--- a/build/gulpfile.hygiene.js
+++ b/build/gulpfile.hygiene.js
@@ -8,10 +8,8 @@
const gulp = require('gulp');
const filter = require('gulp-filter');
const es = require('event-stream');
-const gulptslint = require('gulp-tslint');
const gulpeslint = require('gulp-eslint');
const tsfmt = require('typescript-formatter');
-const tslint = require('tslint');
const VinylFile = require('vinyl');
const vfs = require('vinyl-fs');
const path = require('path');
@@ -35,6 +33,7 @@ const all = [
'scripts/**/*',
'src/**/*',
'test/**/*',
+ '!test/**/out/**',
'!**/node_modules/**'
];
@@ -42,8 +41,8 @@ const indentationFilter = [
'**',
// except specific files
- '!ThirdPartyNotices.txt',
- '!LICENSE.{txt,rtf}',
+ '!**/ThirdPartyNotices.txt',
+ '!**/LICENSE.{txt,rtf}',
'!LICENSES.chromium.html',
'!**/LICENSE',
'!src/vs/nls.js',
@@ -55,11 +54,12 @@ const indentationFilter = [
'!src/vs/base/common/marked/marked.js',
'!src/vs/base/node/terminateProcess.sh',
'!src/vs/base/node/cpuUsage.sh',
- '!test/assert.js',
+ '!test/unit/assert.js',
// except specific folders
'!test/automation/out/**',
'!test/smoke/out/**',
+ '!extensions/typescript-language-features/test-workspace/**',
'!extensions/vscode-api-tests/testWorkspace/**',
'!extensions/vscode-api-tests/testWorkspace2/**',
'!build/monaco/**',
@@ -84,8 +84,8 @@ const indentationFilter = [
'!src/vs/*/**/*.d.ts',
'!src/typings/**/*.d.ts',
'!extensions/**/*.d.ts',
- '!**/*.{svg,exe,png,bmp,scpt,bat,cmd,cur,ttf,woff,eot,md,ps1,template,yaml,yml,d.ts.recipe,ico,icns}',
- '!build/{lib,tslintRules,download}/**/*.js',
+ '!**/*.{svg,exe,png,bmp,scpt,bat,cmd,cur,ttf,woff,eot,md,ps1,template,yaml,yml,d.ts.recipe,ico,icns,plist}',
+ '!build/{lib,download,darwin}/**/*.js',
'!build/**/*.sh',
'!build/azure-pipelines/**/*.js',
'!build/azure-pipelines/**/*.config',
@@ -115,20 +115,19 @@ const copyrightFilter = [
'!**/*.disabled',
'!**/*.code-workspace',
'!**/*.js.map',
- '!**/promise-polyfill/polyfill.js',
'!build/**/*.init',
'!resources/linux/snap/snapcraft.yaml',
'!resources/linux/snap/electron-launch',
'!resources/win32/bin/code.js',
+ '!resources/web/code-web.js',
'!resources/completions/**',
'!extensions/markdown-language-features/media/highlight.css',
'!extensions/html-language-features/server/src/modes/typescript/*',
'!extensions/*/server/bin/*',
- '!src/vs/editor/test/node/classification/typescript-test.ts',
- '!scripts/code-web.js'
+ '!src/vs/editor/test/node/classification/typescript-test.ts'
];
-const eslintFilter = [
+const jsHygieneFilter = [
'src/**/*.js',
'build/gulpfile.*.js',
'!src/vs/loader.js',
@@ -141,7 +140,10 @@ const eslintFilter = [
'!**/test/**'
];
-const tslintBaseFilter = [
+const tsHygieneFilter = [
+ 'src/**/*.ts',
+ 'test/**/*.ts',
+ 'extensions/**/*.ts',
'!**/fixtures/**',
'!**/typings/**',
'!**/node_modules/**',
@@ -152,30 +154,6 @@ const tslintBaseFilter = [
'!extensions/html-language-features/server/lib/jquery.d.ts'
];
-const tslintCoreFilter = [
- 'src/**/*.ts',
- 'test/**/*.ts',
- '!extensions/**/*.ts',
- '!test/automation/**',
- '!test/smoke/**',
- ...tslintBaseFilter
-];
-
-const tslintExtensionsFilter = [
- 'extensions/**/*.ts',
- '!src/**/*.ts',
- '!test/**/*.ts',
- 'test/automation/**/*.ts',
- ...tslintBaseFilter
-];
-
-const tslintHygieneFilter = [
- 'src/**/*.ts',
- 'test/**/*.ts',
- 'extensions/**/*.ts',
- ...tslintBaseFilter
-];
-
const copyrightHeaderLines = [
'/*---------------------------------------------------------------------------------------------',
' * Copyright (c) Microsoft Corporation. All rights reserved.',
@@ -185,27 +163,17 @@ const copyrightHeaderLines = [
gulp.task('eslint', () => {
return vfs.src(all, { base: '.', follow: true, allowEmpty: true })
- .pipe(filter(eslintFilter))
- .pipe(gulpeslint('src/.eslintrc'))
+ .pipe(filter(jsHygieneFilter.concat(tsHygieneFilter)))
+ .pipe(gulpeslint({
+ configFile: '.eslintrc.json',
+ rulePaths: ['./build/lib/eslint']
+ }))
.pipe(gulpeslint.formatEach('compact'))
- .pipe(gulpeslint.failAfterError());
-});
-
-gulp.task('tslint', () => {
- return es.merge([
-
- // Core: include type information (required by certain rules like no-nodejs-globals)
- vfs.src(all, { base: '.', follow: true, allowEmpty: true })
- .pipe(filter(tslintCoreFilter))
- .pipe(gulptslint.default({ rulesDirectory: 'build/lib/tslint', program: tslint.Linter.createProgram('src/tsconfig.json') }))
- .pipe(gulptslint.default.report({ emitError: true })),
-
- // Exenstions: do not include type information
- vfs.src(all, { base: '.', follow: true, allowEmpty: true })
- .pipe(filter(tslintExtensionsFilter))
- .pipe(gulptslint.default({ rulesDirectory: 'build/lib/tslint' }))
- .pipe(gulptslint.default.report({ emitError: true }))
- ]).pipe(es.through());
+ .pipe(gulpeslint.results(results => {
+ if (results.warningCount > 0 || results.errorCount > 0) {
+ throw new Error('eslint failed with warnings and/or errors');
+ }
+ }));
});
function checkPackageJSON(actualPath) {
@@ -227,7 +195,7 @@ function checkPackageJSON(actualPath) {
const checkPackageJSONTask = task.define('check-package-json', () => {
return gulp.src('package.json')
- .pipe(es.through(function() {
+ .pipe(es.through(function () {
checkPackageJSON.call(this, 'remote/package.json');
checkPackageJSON.call(this, 'remote/web/package.json');
}));
@@ -294,8 +262,6 @@ function hygiene(some) {
replace: undefined,
tsconfig: undefined,
tsconfigFile: undefined,
- tslint: undefined,
- tslintFile: undefined,
tsfmtFile: undefined,
vscode: undefined,
vscodeFile: undefined
@@ -304,7 +270,7 @@ function hygiene(some) {
let formatted = result.dest.replace(/\r\n/gm, '\n');
if (original !== formatted) {
- console.error("File not formatted. Run the 'Format Document' command to fix it:", file.relative);
+ console.error('File not formatted. Run the \'Format Document\' command to fix it:', file.relative);
errorCount++;
}
cb(null, file);
@@ -314,20 +280,15 @@ function hygiene(some) {
});
});
- const tslintConfiguration = tslint.Configuration.findConfiguration('tslint.json', '.');
- const tslintOptions = { fix: false, formatter: 'json' };
- const tsLinter = new tslint.Linter(tslintOptions);
-
- const tsl = es.through(function (file) {
- const contents = file.contents.toString('utf8');
- tsLinter.lint(file.relative, contents, tslintConfiguration.results);
- this.emit('data', file);
- });
-
let input;
if (Array.isArray(some) || typeof some === 'string' || !some) {
- input = vfs.src(some || all, { base: '.', follow: true, allowEmpty: true });
+ const options = { base: '.', follow: true, allowEmpty: true };
+ if (some) {
+ input = vfs.src(some, options).pipe(filter(all)); // split this up to not unnecessarily filter all a second time
+ } else {
+ input = vfs.src(all, options);
+ }
} else {
input = some;
}
@@ -344,19 +305,21 @@ function hygiene(some) {
.pipe(filter(copyrightFilter))
.pipe(copyrights);
- let typescript = result
- .pipe(filter(tslintHygieneFilter))
+ const typescript = result
+ .pipe(filter(tsHygieneFilter))
.pipe(formatting);
- if (!process.argv.some(arg => arg === '--skip-tslint')) {
- typescript = typescript.pipe(tsl);
- }
-
const javascript = result
- .pipe(filter(eslintFilter))
- .pipe(gulpeslint('src/.eslintrc'))
+ .pipe(filter(jsHygieneFilter.concat(tsHygieneFilter)))
+ .pipe(gulpeslint({
+ configFile: '.eslintrc.json',
+ rulePaths: ['./build/lib/eslint']
+ }))
.pipe(gulpeslint.formatEach('compact'))
- .pipe(gulpeslint.failAfterError());
+ .pipe(gulpeslint.results(results => {
+ errorCount += results.warningCount;
+ errorCount += results.errorCount;
+ }));
let count = 0;
return es.merge(typescript, javascript)
@@ -368,20 +331,6 @@ function hygiene(some) {
this.emit('data', data);
}, function () {
process.stdout.write('\n');
-
- const tslintResult = tsLinter.getResult();
- if (tslintResult.failures.length > 0) {
- for (const failure of tslintResult.failures) {
- const name = failure.getFileName();
- const position = failure.getStartPosition();
- const line = position.getLineAndCharacter().line;
- const character = position.getLineAndCharacter().character;
-
- console.error(`${name}:${line + 1}:${character + 1}:${failure.getFailure()}`);
- }
- errorCount += tslintResult.failures.length;
- }
-
if (errorCount > 0) {
this.emit('error', 'Hygiene failed with ' + errorCount + ' errors. Check \'build/gulpfile.hygiene.js\'.');
} else {
diff --git a/build/gulpfile.reh.js b/build/gulpfile.reh.js
index a956fba979ef6..5f367d1f0777d 100644
--- a/build/gulpfile.reh.js
+++ b/build/gulpfile.reh.js
@@ -37,19 +37,11 @@ const BUILD_TARGETS = [
const noop = () => { return Promise.resolve(); };
-gulp.task('vscode-reh-win32-ia32-min', noop);
-gulp.task('vscode-reh-win32-x64-min', noop);
-gulp.task('vscode-reh-darwin-min', noop);
-gulp.task('vscode-reh-linux-x64-min', noop);
-gulp.task('vscode-reh-linux-armhf-min', noop);
-gulp.task('vscode-reh-linux-arm64-min', noop);
-gulp.task('vscode-reh-linux-alpine-min', noop);
-
-gulp.task('vscode-reh-web-win32-ia32-min', noop);
-gulp.task('vscode-reh-web-win32-x64-min', noop);
-gulp.task('vscode-reh-web-darwin-min', noop);
-gulp.task('vscode-reh-web-linux-x64-min', noop);
-gulp.task('vscode-reh-web-linux-alpine-min', noop);
+BUILD_TARGETS.forEach(({ platform, arch }) => {
+ for (const target of ['reh', 'reh-web']) {
+ gulp.task(`vscode-${target}-${platform}${ arch ? `-${arch}` : '' }-min`, noop);
+ }
+});
function getNodeVersion() {
const yarnrc = fs.readFileSync(path.join(REPO_ROOT, 'remote', '.yarnrc'), 'utf8');
@@ -118,7 +110,7 @@ function mixinServer(watch) {
const packageJSONPath = path.join(path.dirname(__dirname), 'package.json');
function exec(cmdLine) {
console.log(cmdLine);
- cp.execSync(cmdLine, { stdio: "inherit" });
+ cp.execSync(cmdLine, { stdio: 'inherit' });
}
function checkout() {
const packageJSON = JSON.parse(fs.readFileSync(packageJSONPath).toString());
diff --git a/build/gulpfile.vscode.js b/build/gulpfile.vscode.js
index 93a943aba0be0..d046aa71ed2f2 100644
--- a/build/gulpfile.vscode.js
+++ b/build/gulpfile.vscode.js
@@ -37,18 +37,13 @@ const { compileBuildTask } = require('./gulpfile.compile');
const { compileExtensionsBuildTask } = require('./gulpfile.extensions');
const productionDependencies = deps.getProductionDependencies(path.dirname(__dirname));
-// @ts-ignore
-const baseModules = Object.keys(process.binding('natives')).filter(n => !/^_|\//.test(n));
-const nodeModules = ['electron', 'original-fs']
- // @ts-ignore JSON checking: dependencies property is optional
- .concat(Object.keys(product.dependencies || {}))
- .concat(_.uniq(productionDependencies.map(d => d.name)))
- .concat(baseModules);
// Build
const vscodeEntryPoints = _.flatten([
buildfile.entrypoint('vs/workbench/workbench.desktop.main'),
buildfile.base,
+ buildfile.workerExtensionHost,
+ buildfile.workerNotebook,
buildfile.workbenchDesktop,
buildfile.code
]);
@@ -60,27 +55,32 @@ const vscodeResources = [
'out-build/bootstrap.js',
'out-build/bootstrap-fork.js',
'out-build/bootstrap-amd.js',
+ 'out-build/bootstrap-node.js',
'out-build/bootstrap-window.js',
'out-build/paths.js',
'out-build/vs/**/*.{svg,png,html}',
'!out-build/vs/code/browser/**/*.html',
+ '!out-build/vs/editor/standalone/**/*.svg',
'out-build/vs/base/common/performance.js',
'out-build/vs/base/node/languagePacks.js',
'out-build/vs/base/node/{stdForkStart.js,terminateProcess.sh,cpuUsage.sh,ps.sh}',
- 'out-build/vs/base/browser/ui/codiconLabel/codicon/**',
+ 'out-build/vs/base/browser/ui/codicons/codicon/**',
+ 'out-build/vs/base/parts/sandbox/electron-browser/preload.js',
'out-build/vs/workbench/browser/media/*-theme.css',
'out-build/vs/workbench/contrib/debug/**/*.json',
'out-build/vs/workbench/contrib/externalTerminal/**/*.scpt',
'out-build/vs/workbench/contrib/webview/browser/pre/*.js',
'out-build/vs/workbench/contrib/webview/electron-browser/pre/*.js',
+ 'out-build/vs/workbench/services/extensions/worker/extensionHostWorkerMain.js',
'out-build/vs/**/markdown.css',
'out-build/vs/workbench/contrib/tasks/**/*.json',
'out-build/vs/platform/files/**/*.exe',
'out-build/vs/platform/files/**/*.md',
'out-build/vs/code/electron-browser/workbench/**',
'out-build/vs/code/electron-browser/sharedProcess/sharedProcess.js',
- 'out-build/vs/code/electron-browser/issue/issueReporter.js',
- 'out-build/vs/code/electron-browser/processExplorer/processExplorer.js',
+ 'out-build/vs/code/electron-sandbox/issue/issueReporter.js',
+ 'out-build/vs/code/electron-sandbox/processExplorer/processExplorer.js',
+ 'out-build/vs/code/electron-sandbox/proxy/auth.js',
'!**/test/**'
];
@@ -90,9 +90,8 @@ const optimizeVSCodeTask = task.define('optimize-vscode', task.series(
src: 'out-build',
entryPoints: vscodeEntryPoints,
resources: vscodeResources,
- loaderConfig: common.loaderConfig(nodeModules),
+ loaderConfig: common.loaderConfig(),
out: 'out-vscode',
- inlineAmdImages: true,
bundleInfo: undefined
})
));
@@ -102,12 +101,6 @@ const sourceMappingURLBase = `https://ticino.blob.core.windows.net/sourcemaps/${
const minifyVSCodeTask = task.define('minify-vscode', task.series(
optimizeVSCodeTask,
util.rimraf('out-vscode-min'),
- () => {
- const fullpath = path.join(process.cwd(), 'out-vscode/bootstrap-window.js');
- const contents = fs.readFileSync(fullpath).toString();
- const newContents = contents.replace('[/*BUILD->INSERT_NODE_MODULES*/]', JSON.stringify(nodeModules));
- fs.writeFileSync(fullpath, newContents);
- },
common.minifyTask('out-vscode', `${sourceMappingURLBase}/core`)
));
gulp.task(minifyVSCodeTask);
@@ -120,9 +113,9 @@ gulp.task(minifyVSCodeTask);
* @return {Object} A map of paths to checksums.
*/
function computeChecksums(out, filenames) {
- var result = {};
+ let result = {};
filenames.forEach(function (filename) {
- var fullPath = path.join(process.cwd(), out, filename);
+ let fullPath = path.join(process.cwd(), out, filename);
result[filename] = computeChecksum(fullPath);
});
return result;
@@ -135,9 +128,9 @@ function computeChecksums(out, filenames) {
* @return {string} The checksum for `filename`.
*/
function computeChecksum(filename) {
- var contents = fs.readFileSync(filename);
+ let contents = fs.readFileSync(filename);
- var hash = crypto
+ let hash = crypto
.createHash('md5')
.update(contents)
.digest('base64')
@@ -156,8 +149,10 @@ function packageTask(platform, arch, sourceFolderName, destinationFolderName, op
const out = sourceFolderName;
const checksums = computeChecksums(out, [
+ 'vs/base/parts/sandbox/electron-browser/preload.js',
'vs/workbench/workbench.desktop.main.js',
'vs/workbench/workbench.desktop.main.css',
+ 'vs/workbench/services/extensions/node/extensionHostProcess.js',
'vs/code/electron-browser/workbench/workbench.html',
'vs/code/electron-browser/workbench/workbench.js'
]);
@@ -212,7 +207,7 @@ function packageTask(platform, arch, sourceFolderName, destinationFolderName, op
const deps = gulp.src(dependenciesSrc, { base: '.', dot: true })
.pipe(filter(['**', '!**/package-lock.json']))
.pipe(util.cleanNodeModules(path.join(__dirname, '.nativeignore')))
- .pipe(createAsar(path.join(process.cwd(), 'node_modules'), ['**/*.node', '**/vscode-ripgrep/bin/*', '**/node-pty/build/Release/*'], 'app/node_modules.asar'));
+ .pipe(createAsar(path.join(process.cwd(), 'node_modules'), ['**/*.node', '**/vscode-ripgrep/bin/*', '**/node-pty/build/Release/*', '**/*.wasm'], 'app/node_modules.asar'));
let all = es.merge(
packageJsonStream,
@@ -268,7 +263,7 @@ function packageTask(platform, arch, sourceFolderName, destinationFolderName, op
let result = all
.pipe(util.skipDirectories())
.pipe(util.fixWin32DirectoryPermissions())
- .pipe(electron(_.extend({}, config, { platform, arch, ffmpegChromium: true })))
+ .pipe(electron(_.extend({}, config, { platform, arch: arch === 'armhf' ? 'arm' : arch, ffmpegChromium: true })))
.pipe(filter(['**', '!LICENSE', '!LICENSES.chromium.html', '!version'], { dot: true }));
if (platform === 'linux') {
@@ -325,10 +320,11 @@ const buildRoot = path.dirname(root);
const BUILD_TARGETS = [
{ platform: 'win32', arch: 'ia32' },
{ platform: 'win32', arch: 'x64' },
+ { platform: 'win32', arch: 'arm64' },
{ platform: 'darwin', arch: null, opts: { stats: true } },
{ platform: 'linux', arch: 'ia32' },
{ platform: 'linux', arch: 'x64' },
- { platform: 'linux', arch: 'arm' },
+ { platform: 'linux', arch: 'armhf' },
{ platform: 'linux', arch: 'arm64' },
];
BUILD_TARGETS.forEach(buildTarget => {
@@ -427,7 +423,7 @@ gulp.task('vscode-translations-pull', function () {
});
gulp.task('vscode-translations-import', function () {
- var options = minimist(process.argv.slice(2), {
+ let options = minimist(process.argv.slice(2), {
string: 'location',
default: {
location: '../vscode-translations-import'
@@ -457,20 +453,30 @@ const generateVSCodeConfigurationTask = task.define('generate-vscode-configurati
const extensionsDir = path.join(os.tmpdir(), 'tmpextdir');
const appName = process.env.VSCODE_QUALITY === 'insider' ? 'Visual\\ Studio\\ Code\\ -\\ Insiders.app' : 'Visual\\ Studio\\ Code.app';
const appPath = path.join(buildDir, `VSCode-darwin/${appName}/Contents/Resources/app/bin/code`);
- const codeProc = cp.exec(`${appPath} --export-default-configuration='${allConfigDetailsPath}' --wait --user-data-dir='${userDataDir}' --extensions-dir='${extensionsDir}'`);
-
+ const codeProc = cp.exec(
+ `${appPath} --export-default-configuration='${allConfigDetailsPath}' --wait --user-data-dir='${userDataDir}' --extensions-dir='${extensionsDir}'`,
+ (err, stdout, stderr) => {
+ clearTimeout(timer);
+ if (err) {
+ console.log(`err: ${err} ${err.message} ${err.toString()}`);
+ reject(err);
+ }
+
+ if (stdout) {
+ console.log(`stdout: ${stdout}`);
+ }
+
+ if (stderr) {
+ console.log(`stderr: ${stderr}`);
+ }
+
+ resolve();
+ }
+ );
const timer = setTimeout(() => {
codeProc.kill();
reject(new Error('export-default-configuration process timed out'));
- }, 10 * 1000);
-
- codeProc.stdout.on('data', d => console.log(d.toString()));
- codeProc.stderr.on('data', d => console.log(d.toString()));
-
- codeProc.on('exit', () => {
- clearTimeout(timer);
- resolve();
- });
+ }, 12 * 1000);
codeProc.on('error', err => {
clearTimeout(timer);
diff --git a/build/gulpfile.vscode.linux.js b/build/gulpfile.vscode.linux.js
index 9d3a7caa07e68..1d8a09e4fe617 100644
--- a/build/gulpfile.vscode.linux.js
+++ b/build/gulpfile.vscode.linux.js
@@ -23,7 +23,7 @@ const commit = util.getVersion(root);
const linuxPackageRevision = Math.floor(new Date().getTime() / 1000);
function getDebPackageArch(arch) {
- return { x64: 'amd64', arm: 'armhf', arm64: "arm64" }[arch];
+ return { x64: 'amd64', armhf: 'armhf', arm64: 'arm64' }[arch];
}
function prepareDebPackage(arch) {
@@ -43,7 +43,7 @@ function prepareDebPackage(arch) {
.pipe(replace('@@NAME_SHORT@@', product.nameShort))
.pipe(replace('@@NAME@@', product.applicationName))
.pipe(replace('@@EXEC@@', `/usr/share/${product.applicationName}/${product.applicationName}`))
- .pipe(replace('@@ICON@@', `/usr/share/pixmaps/${product.linuxIconName}.png`))
+ .pipe(replace('@@ICON@@', product.linuxIconName))
.pipe(replace('@@URLPROTOCOL@@', product.urlProtocol));
const appdata = gulp.src('resources/linux/code.appdata.xml', { base: '.' })
@@ -52,6 +52,11 @@ function prepareDebPackage(arch) {
.pipe(replace('@@LICENSE@@', product.licenseName))
.pipe(rename('usr/share/appdata/' + product.applicationName + '.appdata.xml'));
+ const workspaceMime = gulp.src('resources/linux/code-workspace.xml', { base: '.' })
+ .pipe(replace('@@NAME_LONG@@', product.nameLong))
+ .pipe(replace('@@NAME@@', product.applicationName))
+ .pipe(rename('usr/share/mime/packages/' + product.applicationName + '-workspace.xml'));
+
const icon = gulp.src('resources/linux/code.png', { base: '.' })
.pipe(rename('usr/share/pixmaps/' + product.linuxIconName + '.png'));
@@ -91,13 +96,11 @@ function prepareDebPackage(arch) {
const postinst = gulp.src('resources/linux/debian/postinst.template', { base: '.' })
.pipe(replace('@@NAME@@', product.applicationName))
.pipe(replace('@@ARCHITECTURE@@', debArch))
- // @ts-ignore JSON checking: quality is optional
.pipe(replace('@@QUALITY@@', product.quality || '@@QUALITY@@'))
- // @ts-ignore JSON checking: updateUrl is optional
.pipe(replace('@@UPDATEURL@@', product.updateUrl || '@@UPDATEURL@@'))
.pipe(rename('DEBIAN/postinst'));
- const all = es.merge(control, postinst, postrm, prerm, desktops, appdata, icon, bash_completion, zsh_completion, code);
+ const all = es.merge(control, postinst, postrm, prerm, desktops, appdata, workspaceMime, icon, bash_completion, zsh_completion, code);
return all.pipe(vfs.dest(destination));
};
@@ -117,7 +120,7 @@ function getRpmBuildPath(rpmArch) {
}
function getRpmPackageArch(arch) {
- return { x64: 'x86_64', arm: 'armhf', arm64: "arm64" }[arch];
+ return { x64: 'x86_64', armhf: 'armv7hl', arm64: 'aarch64' }[arch];
}
function prepareRpmPackage(arch) {
@@ -145,6 +148,11 @@ function prepareRpmPackage(arch) {
.pipe(replace('@@LICENSE@@', product.licenseName))
.pipe(rename('usr/share/appdata/' + product.applicationName + '.appdata.xml'));
+ const workspaceMime = gulp.src('resources/linux/code-workspace.xml', { base: '.' })
+ .pipe(replace('@@NAME_LONG@@', product.nameLong))
+ .pipe(replace('@@NAME@@', product.applicationName))
+ .pipe(rename('BUILD/usr/share/mime/packages/' + product.applicationName + '-workspace.xml'));
+
const icon = gulp.src('resources/linux/code.png', { base: '.' })
.pipe(rename('BUILD/usr/share/pixmaps/' + product.linuxIconName + '.png'));
@@ -167,9 +175,7 @@ function prepareRpmPackage(arch) {
.pipe(replace('@@RELEASE@@', linuxPackageRevision))
.pipe(replace('@@ARCHITECTURE@@', rpmArch))
.pipe(replace('@@LICENSE@@', product.licenseName))
- // @ts-ignore JSON checking: quality is optional
.pipe(replace('@@QUALITY@@', product.quality || '@@QUALITY@@'))
- // @ts-ignore JSON checking: updateUrl is optional
.pipe(replace('@@UPDATEURL@@', product.updateUrl || '@@UPDATEURL@@'))
.pipe(replace('@@DEPENDENCIES@@', rpmDependencies[rpmArch].join(', ')))
.pipe(rename('SPECS/' + product.applicationName + '.spec'));
@@ -177,7 +183,7 @@ function prepareRpmPackage(arch) {
const specIcon = gulp.src('resources/linux/rpm/code.xpm', { base: '.' })
.pipe(rename('SOURCES/' + product.applicationName + '.xpm'));
- const all = es.merge(code, desktops, appdata, icon, bash_completion, zsh_completion, spec, specIcon);
+ const all = es.merge(code, desktops, appdata, workspaceMime, icon, bash_completion, zsh_completion, spec, specIcon);
return all.pipe(vfs.dest(getRpmBuildPath(rpmArch)));
};
@@ -250,33 +256,23 @@ function buildSnapPackage(arch) {
const BUILD_TARGETS = [
{ arch: 'x64' },
- { arch: 'arm' },
+ { arch: 'armhf' },
{ arch: 'arm64' },
];
-BUILD_TARGETS.forEach((buildTarget) => {
- const arch = buildTarget.arch;
-
- {
- const debArch = getDebPackageArch(arch);
- const prepareDebTask = task.define(`vscode-linux-${arch}-prepare-deb`, task.series(util.rimraf(`.build/linux/deb/${debArch}`), prepareDebPackage(arch)));
- // gulp.task(prepareDebTask);
- const buildDebTask = task.define(`vscode-linux-${arch}-build-deb`, task.series(prepareDebTask, buildDebPackage(arch)));
- gulp.task(buildDebTask);
- }
-
- {
- const rpmArch = getRpmPackageArch(arch);
- const prepareRpmTask = task.define(`vscode-linux-${arch}-prepare-rpm`, task.series(util.rimraf(`.build/linux/rpm/${rpmArch}`), prepareRpmPackage(arch)));
- // gulp.task(prepareRpmTask);
- const buildRpmTask = task.define(`vscode-linux-${arch}-build-rpm`, task.series(prepareRpmTask, buildRpmPackage(arch)));
- gulp.task(buildRpmTask);
- }
-
- {
- const prepareSnapTask = task.define(`vscode-linux-${arch}-prepare-snap`, task.series(util.rimraf(`.build/linux/snap/${arch}`), prepareSnapPackage(arch)));
- gulp.task(prepareSnapTask);
- const buildSnapTask = task.define(`vscode-linux-${arch}-build-snap`, task.series(prepareSnapTask, buildSnapPackage(arch)));
- gulp.task(buildSnapTask);
- }
+BUILD_TARGETS.forEach(({ arch }) => {
+ const debArch = getDebPackageArch(arch);
+ const prepareDebTask = task.define(`vscode-linux-${arch}-prepare-deb`, task.series(util.rimraf(`.build/linux/deb/${debArch}`), prepareDebPackage(arch)));
+ const buildDebTask = task.define(`vscode-linux-${arch}-build-deb`, task.series(prepareDebTask, buildDebPackage(arch)));
+ gulp.task(buildDebTask);
+
+ const rpmArch = getRpmPackageArch(arch);
+ const prepareRpmTask = task.define(`vscode-linux-${arch}-prepare-rpm`, task.series(util.rimraf(`.build/linux/rpm/${rpmArch}`), prepareRpmPackage(arch)));
+ const buildRpmTask = task.define(`vscode-linux-${arch}-build-rpm`, task.series(prepareRpmTask, buildRpmPackage(arch)));
+ gulp.task(buildRpmTask);
+
+ const prepareSnapTask = task.define(`vscode-linux-${arch}-prepare-snap`, task.series(util.rimraf(`.build/linux/snap/${arch}`), prepareSnapPackage(arch)));
+ gulp.task(prepareSnapTask);
+ const buildSnapTask = task.define(`vscode-linux-${arch}-build-snap`, task.series(prepareSnapTask, buildSnapPackage(arch)));
+ gulp.task(buildSnapTask);
});
diff --git a/build/gulpfile.vscode.win32.js b/build/gulpfile.vscode.win32.js
index 497fc553c038a..2abc39976b42f 100644
--- a/build/gulpfile.vscode.win32.js
+++ b/build/gulpfile.vscode.win32.js
@@ -65,6 +65,7 @@ function buildWin32Setup(arch, target) {
return cb => {
const ia32AppId = target === 'system' ? product.win32AppId : product.win32UserAppId;
const x64AppId = target === 'system' ? product.win32x64AppId : product.win32x64UserAppId;
+ const arm64AppId = target === 'system' ? product.win32arm64AppId : product.win32arm64UserAppId;
const sourcePath = buildPath(arch);
const outputPath = setupDir(arch, target);
@@ -88,12 +89,12 @@ function buildWin32Setup(arch, target) {
ShellNameShort: product.win32ShellNameShort,
AppMutex: product.win32MutexName,
Arch: arch,
- AppId: arch === 'ia32' ? ia32AppId : x64AppId,
- IncompatibleTargetAppId: arch === 'ia32' ? product.win32AppId : product.win32x64AppId,
- IncompatibleArchAppId: arch === 'ia32' ? x64AppId : ia32AppId,
+ AppId: { 'ia32': ia32AppId, 'x64': x64AppId, 'arm64': arm64AppId }[arch],
+ IncompatibleTargetAppId: { 'ia32': product.win32AppId, 'x64': product.win32x64AppId, 'arm64': product.win32arm64AppId }[arch],
+ IncompatibleArchAppId: { 'ia32': x64AppId, 'x64': ia32AppId, 'arm64': ia32AppId }[arch],
AppUserId: product.win32AppUserModelId,
- ArchitecturesAllowed: arch === 'ia32' ? '' : 'x64',
- ArchitecturesInstallIn64BitMode: arch === 'ia32' ? '' : 'x64',
+ ArchitecturesAllowed: { 'ia32': '', 'x64': 'x64', 'arm64': 'arm64' }[arch],
+ ArchitecturesInstallIn64BitMode: { 'ia32': '', 'x64': 'x64', 'arm64': 'arm64' }[arch],
SourceDir: sourcePath,
RepoDir: repoPath,
OutputDir: outputPath,
@@ -112,8 +113,10 @@ function defineWin32SetupTasks(arch, target) {
defineWin32SetupTasks('ia32', 'system');
defineWin32SetupTasks('x64', 'system');
+defineWin32SetupTasks('arm64', 'system');
defineWin32SetupTasks('ia32', 'user');
defineWin32SetupTasks('x64', 'user');
+defineWin32SetupTasks('arm64', 'user');
function archiveWin32Setup(arch) {
return cb => {
@@ -127,6 +130,7 @@ function archiveWin32Setup(arch) {
gulp.task(task.define('vscode-win32-ia32-archive', task.series(util.rimraf(zipDir('ia32')), archiveWin32Setup('ia32'))));
gulp.task(task.define('vscode-win32-x64-archive', task.series(util.rimraf(zipDir('x64')), archiveWin32Setup('x64'))));
+gulp.task(task.define('vscode-win32-arm64-archive', task.series(util.rimraf(zipDir('arm64')), archiveWin32Setup('arm64'))));
function copyInnoUpdater(arch) {
return () => {
@@ -144,8 +148,10 @@ function updateIcon(executablePath) {
gulp.task(task.define('vscode-win32-ia32-inno-updater', task.series(copyInnoUpdater('ia32'), updateIcon(path.join(buildPath('ia32'), 'tools', 'inno_updater.exe')))));
gulp.task(task.define('vscode-win32-x64-inno-updater', task.series(copyInnoUpdater('x64'), updateIcon(path.join(buildPath('x64'), 'tools', 'inno_updater.exe')))));
+gulp.task(task.define('vscode-win32-arm64-inno-updater', task.series(copyInnoUpdater('arm64'), updateIcon(path.join(buildPath('arm64'), 'tools', 'inno_updater.exe')))));
// CodeHelper.exe icon
gulp.task(task.define('vscode-win32-ia32-code-helper', task.series(updateIcon(path.join(buildPath('ia32'), 'resources', 'app', 'out', 'vs', 'platform', 'files', 'node', 'watcher', 'win32', 'CodeHelper.exe')))));
gulp.task(task.define('vscode-win32-x64-code-helper', task.series(updateIcon(path.join(buildPath('x64'), 'resources', 'app', 'out', 'vs', 'platform', 'files', 'node', 'watcher', 'win32', 'CodeHelper.exe')))));
+gulp.task(task.define('vscode-win32-arm64-code-helper', task.series(updateIcon(path.join(buildPath('arm64'), 'resources', 'app', 'out', 'vs', 'platform', 'files', 'node', 'watcher', 'win32', 'CodeHelper.exe')))));
diff --git a/build/lib/asar.js b/build/lib/asar.js
index 21c5f65a45b06..f48583c0b46c6 100644
--- a/build/lib/asar.js
+++ b/build/lib/asar.js
@@ -4,6 +4,7 @@
*--------------------------------------------------------------------------------------------*/
'use strict';
Object.defineProperty(exports, "__esModule", { value: true });
+exports.createAsar = void 0;
const path = require("path");
const es = require("event-stream");
const pickle = require('chromium-pickle-js');
@@ -52,7 +53,9 @@ function createAsar(folderPath, unpackGlobs, destFilename) {
const insertFile = (relativePath, stat, shouldUnpack) => {
insertDirectoryForFile(relativePath);
pendingInserts++;
- filesystem.insertFile(relativePath, shouldUnpack, { stat: stat }, {}, onFileInserted);
+ // Do not pass `onFileInserted` directly because it gets overwritten below.
+ // Create a closure capturing `onFileInserted`.
+ filesystem.insertFile(relativePath, shouldUnpack, { stat: stat }, {}).then(() => onFileInserted(), () => onFileInserted());
};
return es.through(function (file) {
if (file.stat.isDirectory()) {
diff --git a/build/lib/asar.ts b/build/lib/asar.ts
index d2823043aabfc..38fbc634e990d 100644
--- a/build/lib/asar.ts
+++ b/build/lib/asar.ts
@@ -8,10 +8,17 @@
import * as path from 'path';
import * as es from 'event-stream';
const pickle = require('chromium-pickle-js');
-const Filesystem = require('asar/lib/filesystem');
+const Filesystem = require('asar/lib/filesystem');
import * as VinylFile from 'vinyl';
import * as minimatch from 'minimatch';
+declare class AsarFilesystem {
+ readonly header: unknown;
+ constructor(src: string);
+ insertDirectory(path: string, shouldUnpack?: boolean): unknown;
+ insertFile(path: string, shouldUnpack: boolean, file: { stat: { size: number; mode: number; }; }, options: {}): Promise;
+}
+
export function createAsar(folderPath: string, unpackGlobs: string[], destFilename: string): NodeJS.ReadWriteStream {
const shouldUnpackFile = (file: VinylFile): boolean => {
@@ -61,7 +68,9 @@ export function createAsar(folderPath: string, unpackGlobs: string[], destFilena
const insertFile = (relativePath: string, stat: { size: number; mode: number; }, shouldUnpack: boolean) => {
insertDirectoryForFile(relativePath);
pendingInserts++;
- filesystem.insertFile(relativePath, shouldUnpack, { stat: stat }, {}, onFileInserted);
+ // Do not pass `onFileInserted` directly because it gets overwritten below.
+ // Create a closure capturing `onFileInserted`.
+ filesystem.insertFile(relativePath, shouldUnpack, { stat: stat }, {}).then(() => onFileInserted(), () => onFileInserted());
};
return es.through(function (file) {
diff --git a/build/lib/builtInExtensions.js b/build/lib/builtInExtensions.js
index a687bbccb4cb5..f86414211ec0c 100644
--- a/build/lib/builtInExtensions.js
+++ b/build/lib/builtInExtensions.js
@@ -18,8 +18,17 @@ const fancyLog = require('fancy-log');
const ansiColors = require('ansi-colors');
const root = path.dirname(path.dirname(__dirname));
-const builtInExtensions = require('../builtInExtensions.json');
+const productjson = JSON.parse(fs.readFileSync(path.join(__dirname, '../../product.json'), 'utf8'));
+const builtInExtensions = productjson.builtInExtensions;
+const webBuiltInExtensions = productjson.webBuiltInExtensions;
const controlFilePath = path.join(os.homedir(), '.vscode-oss-dev', 'extensions', 'control.json');
+const ENABLE_LOGGING = !process.env['VSCODE_BUILD_BUILTIN_EXTENSIONS_SILENCE_PLEASE'];
+
+function log() {
+ if (ENABLE_LOGGING) {
+ fancyLog.apply(this, arguments);
+ }
+}
function getExtensionPath(extension) {
return path.join(root, '.build', 'builtInExtensions', extension.name);
@@ -44,7 +53,7 @@ function isUpToDate(extension) {
function syncMarketplaceExtension(extension) {
if (isUpToDate(extension)) {
- fancyLog(ansiColors.blue('[marketplace]'), `${extension.name}@${extension.version}`, ansiColors.green('✔︎'));
+ log(ansiColors.blue('[marketplace]'), `${extension.name}@${extension.version}`, ansiColors.green('✔︎'));
return es.readArray([]);
}
@@ -53,13 +62,13 @@ function syncMarketplaceExtension(extension) {
return ext.fromMarketplace(extension.name, extension.version, extension.metadata)
.pipe(rename(p => p.dirname = `${extension.name}/${p.dirname}`))
.pipe(vfs.dest('.build/builtInExtensions'))
- .on('end', () => fancyLog(ansiColors.blue('[marketplace]'), extension.name, ansiColors.green('✔︎')));
+ .on('end', () => log(ansiColors.blue('[marketplace]'), extension.name, ansiColors.green('✔︎')));
}
function syncExtension(extension, controlState) {
switch (controlState) {
case 'disabled':
- fancyLog(ansiColors.blue('[disabled]'), ansiColors.gray(extension.name));
+ log(ansiColors.blue('[disabled]'), ansiColors.gray(extension.name));
return es.readArray([]);
case 'marketplace':
@@ -67,15 +76,15 @@ function syncExtension(extension, controlState) {
default:
if (!fs.existsSync(controlState)) {
- fancyLog(ansiColors.red(`Error: Built-in extension '${extension.name}' is configured to run from '${controlState}' but that path does not exist.`));
+ log(ansiColors.red(`Error: Built-in extension '${extension.name}' is configured to run from '${controlState}' but that path does not exist.`));
return es.readArray([]);
} else if (!fs.existsSync(path.join(controlState, 'package.json'))) {
- fancyLog(ansiColors.red(`Error: Built-in extension '${extension.name}' is configured to run from '${controlState}' but there is no 'package.json' file in that directory.`));
+ log(ansiColors.red(`Error: Built-in extension '${extension.name}' is configured to run from '${controlState}' but there is no 'package.json' file in that directory.`));
return es.readArray([]);
}
- fancyLog(ansiColors.blue('[local]'), `${extension.name}: ${ansiColors.cyan(controlState)}`, ansiColors.green('✔︎'));
+ log(ansiColors.blue('[local]'), `${extension.name}: ${ansiColors.cyan(controlState)}`, ansiColors.green('✔︎'));
return es.readArray([]);
}
}
@@ -93,14 +102,14 @@ function writeControlFile(control) {
fs.writeFileSync(controlFilePath, JSON.stringify(control, null, 2));
}
-function main() {
- fancyLog('Syncronizing built-in extensions...');
- fancyLog(`You can manage built-in extensions with the ${ansiColors.cyan('--builtin')} flag`);
+exports.getBuiltInExtensions = function getBuiltInExtensions() {
+ log('Syncronizing built-in extensions...');
+ log(`You can manage built-in extensions with the ${ansiColors.cyan('--builtin')} flag`);
const control = readControlFile();
const streams = [];
- for (const extension of builtInExtensions) {
+ for (const extension of [...builtInExtensions, ...webBuiltInExtensions]) {
let controlState = control[extension.name] || 'marketplace';
control[extension.name] = controlState;
@@ -109,14 +118,16 @@ function main() {
writeControlFile(control);
- es.merge(streams)
- .on('error', err => {
- console.error(err);
- process.exit(1);
- })
- .on('end', () => {
- process.exit(0);
- });
+ return new Promise((resolve, reject) => {
+ es.merge(streams)
+ .on('error', reject)
+ .on('end', resolve);
+ });
+};
+
+if (require.main === module) {
+ exports.getBuiltInExtensions().then(() => process.exit(0)).catch(err => {
+ console.error(err);
+ process.exit(1);
+ });
}
-
-main();
diff --git a/build/lib/bundle.js b/build/lib/bundle.js
index 881e8ff6c7f0d..7d0c8d9b55ef0 100644
--- a/build/lib/bundle.js
+++ b/build/lib/bundle.js
@@ -4,6 +4,7 @@
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
Object.defineProperty(exports, "__esModule", { value: true });
+exports.bundle = void 0;
const fs = require("fs");
const path = require("path");
const vm = require("vm");
diff --git a/build/lib/compilation.js b/build/lib/compilation.js
index 170e086b35b7c..07cf31655419e 100644
--- a/build/lib/compilation.js
+++ b/build/lib/compilation.js
@@ -4,6 +4,7 @@
*--------------------------------------------------------------------------------------------*/
'use strict';
Object.defineProperty(exports, "__esModule", { value: true });
+exports.watchTask = exports.compileTask = void 0;
const es = require("event-stream");
const fs = require("fs");
const gulp = require("gulp");
@@ -17,6 +18,7 @@ const reporter_1 = require("./reporter");
const util = require("./util");
const fancyLog = require("fancy-log");
const ansiColors = require("ansi-colors");
+const os = require("os");
const watch = require('./watch');
const reporter = reporter_1.createReporter();
function getTypeScriptCompilerOptions(src) {
@@ -44,7 +46,7 @@ function createCompile(src, build, emitError) {
const input = es.through();
const output = input
.pipe(utf8Filter)
- .pipe(bom())
+ .pipe(bom()) // this is required to preserve BOM in test files that loose it otherwise
.pipe(utf8Filter.restore)
.pipe(tsFilter)
.pipe(util.loadSourcemaps())
@@ -68,6 +70,9 @@ function createCompile(src, build, emitError) {
}
function compileTask(src, out, build) {
return function () {
+ if (os.totalmem() < 4000000000) {
+ throw new Error('compilation requires 4GB of RAM');
+ }
const compile = createCompile(src, build, true);
const srcPipe = gulp.src(`${src}/**`, { base: `${src}` });
let generator = new MonacoGenerator(false);
diff --git a/build/lib/compilation.ts b/build/lib/compilation.ts
index 6f37821c7750b..11a0c7f2da753 100644
--- a/build/lib/compilation.ts
+++ b/build/lib/compilation.ts
@@ -18,6 +18,7 @@ import { createReporter } from './reporter';
import * as util from './util';
import * as fancyLog from 'fancy-log';
import * as ansiColors from 'ansi-colors';
+import * as os from 'os';
import ts = require('typescript');
const watch = require('./watch');
@@ -54,7 +55,7 @@ function createCompile(src: string, build: boolean, emitError?: boolean) {
const input = es.through();
const output = input
.pipe(utf8Filter)
- .pipe(bom())
+ .pipe(bom()) // this is required to preserve BOM in test files that loose it otherwise
.pipe(utf8Filter.restore)
.pipe(tsFilter)
.pipe(util.loadSourcemaps())
@@ -81,6 +82,11 @@ function createCompile(src: string, build: boolean, emitError?: boolean) {
export function compileTask(src: string, out: string, build: boolean): () => NodeJS.ReadWriteStream {
return function () {
+
+ if (os.totalmem() < 4_000_000_000) {
+ throw new Error('compilation requires 4GB of RAM');
+ }
+
const compile = createCompile(src, build, true);
const srcPipe = gulp.src(`${src}/**`, { base: `${src}` });
let generator = new MonacoGenerator(false);
diff --git a/build/lib/electron.js b/build/lib/electron.js
index c7c4cd9a69339..301a769506042 100644
--- a/build/lib/electron.js
+++ b/build/lib/electron.js
@@ -4,6 +4,7 @@
*--------------------------------------------------------------------------------------------*/
'use strict';
Object.defineProperty(exports, "__esModule", { value: true });
+exports.config = void 0;
const fs = require("fs");
const path = require("path");
const vfs = require("vinyl-fs");
@@ -15,12 +16,6 @@ const electron = require('gulp-atom-electron');
const root = path.dirname(path.dirname(__dirname));
const product = JSON.parse(fs.readFileSync(path.join(root, 'product.json'), 'utf8'));
const commit = util.getVersion(root);
-function getElectronVersion() {
- const yarnrc = fs.readFileSync(path.join(root, '.yarnrc'), 'utf8');
- const target = /^target "(.*)"$/m.exec(yarnrc)[1];
- return target;
-}
-exports.getElectronVersion = getElectronVersion;
const darwinCreditsTemplate = product.darwinCredits && _.template(fs.readFileSync(path.join(root, product.darwinCredits), 'utf8'));
function darwinBundleDocumentType(extensions, icon) {
return {
@@ -32,7 +27,7 @@ function darwinBundleDocumentType(extensions, icon) {
};
}
exports.config = {
- version: getElectronVersion(),
+ version: util.getElectronVersion(),
productAppName: product.nameLong,
companyName: 'Microsoft Corporation',
copyright: 'Copyright (C) 2019 Microsoft. All rights reserved',
@@ -46,14 +41,14 @@ exports.config = {
darwinBundleDocumentType(["bowerrc"], 'resources/darwin/bower.icns'),
darwinBundleDocumentType(["c", "h"], 'resources/darwin/c.icns'),
darwinBundleDocumentType(["config", "editorconfig", "gitattributes", "gitconfig", "gitignore", "ini"], 'resources/darwin/config.icns'),
- darwinBundleDocumentType(["cc", "cpp", "cxx", "hh", "hpp", "hxx"], 'resources/darwin/cpp.icns'),
+ darwinBundleDocumentType(["cc", "cpp", "cxx", "c++", "hh", "hpp", "hxx", "h++"], 'resources/darwin/cpp.icns'),
darwinBundleDocumentType(["cs", "csx"], 'resources/darwin/csharp.icns'),
darwinBundleDocumentType(["css"], 'resources/darwin/css.icns'),
darwinBundleDocumentType(["go"], 'resources/darwin/go.icns'),
darwinBundleDocumentType(["asp", "aspx", "cshtml", "htm", "html", "jshtm", "jsp", "phtml", "shtml"], 'resources/darwin/html.icns'),
darwinBundleDocumentType(["jade"], 'resources/darwin/jade.icns'),
darwinBundleDocumentType(["jav", "java"], 'resources/darwin/java.icns'),
- darwinBundleDocumentType(["js", "jscsrc", "jshintrc", "mjs"], 'resources/darwin/javascript.icns'),
+ darwinBundleDocumentType(["js", "jscsrc", "jshintrc", "mjs", "cjs"], 'resources/darwin/javascript.icns'),
darwinBundleDocumentType(["json"], 'resources/darwin/json.icns'),
darwinBundleDocumentType(["less"], 'resources/darwin/less.icns'),
darwinBundleDocumentType(["markdown", "md", "mdoc", "mdown", "mdtext", "mdtxt", "mdwn", "mkd", "mkdn"], 'resources/darwin/markdown.icns'),
@@ -69,7 +64,7 @@ exports.config = {
darwinBundleDocumentType(["vue"], 'resources/darwin/vue.icns'),
darwinBundleDocumentType(["ascx", "csproj", "dtd", "wxi", "wxl", "wxs", "xml", "xaml"], 'resources/darwin/xml.icns'),
darwinBundleDocumentType(["eyaml", "eyml", "yaml", "yml"], 'resources/darwin/yaml.icns'),
- darwinBundleDocumentType(["clj", "cljs", "cljx", "clojure", "code-workspace", "coffee", "ctp", "dockerfile", "dot", "edn", "fs", "fsi", "fsscript", "fsx", "handlebars", "hbs", "lua", "m", "makefile", "ml", "mli", "pl", "pl6", "pm", "pm6", "pod", "pp", "properties", "psgi", "pug", "r", "rs", "rt", "svg", "svgz", "t", "txt", "vb", "xcodeproj", "xcworkspace"], 'resources/darwin/default.icns')
+ darwinBundleDocumentType(["clj", "cljs", "cljx", "clojure", "code-workspace", "coffee", "containerfile", "ctp", "dockerfile", "dot", "edn", "fs", "fsi", "fsscript", "fsx", "handlebars", "hbs", "lua", "m", "makefile", "ml", "mli", "pl", "pl6", "pm", "pm6", "pod", "pp", "properties", "psgi", "pug", "r", "rs", "rt", "svg", "svgz", "t", "txt", "vb", "xcodeproj", "xcworkspace"], 'resources/darwin/default.icns')
],
darwinBundleURLTypes: [{
role: 'Viewer',
@@ -87,7 +82,7 @@ function getElectron(arch) {
return () => {
const electronOpts = _.extend({}, exports.config, {
platform: process.platform,
- arch,
+ arch: arch === 'armhf' ? 'arm' : arch,
ffmpegChromium: true,
keepDefaultApp: true
});
@@ -99,7 +94,7 @@ function getElectron(arch) {
};
}
async function main(arch = process.arch) {
- const version = getElectronVersion();
+ const version = util.getElectronVersion();
const electronPath = path.join(root, '.build', 'electron');
const versionFile = path.join(electronPath, 'version');
const isUpToDate = fs.existsSync(versionFile) && fs.readFileSync(versionFile, 'utf8') === `${version}`;
diff --git a/build/lib/electron.ts b/build/lib/electron.ts
index 90a25f4cac8d9..125759c581562 100644
--- a/build/lib/electron.ts
+++ b/build/lib/electron.ts
@@ -19,12 +19,6 @@ const root = path.dirname(path.dirname(__dirname));
const product = JSON.parse(fs.readFileSync(path.join(root, 'product.json'), 'utf8'));
const commit = util.getVersion(root);
-export function getElectronVersion(): string {
- const yarnrc = fs.readFileSync(path.join(root, '.yarnrc'), 'utf8');
- const target = /^target "(.*)"$/m.exec(yarnrc)![1];
- return target;
-}
-
const darwinCreditsTemplate = product.darwinCredits && _.template(fs.readFileSync(path.join(root, product.darwinCredits), 'utf8'));
function darwinBundleDocumentType(extensions: string[], icon: string) {
@@ -38,7 +32,7 @@ function darwinBundleDocumentType(extensions: string[], icon: string) {
}
export const config = {
- version: getElectronVersion(),
+ version: util.getElectronVersion(),
productAppName: product.nameLong,
companyName: 'Microsoft Corporation',
copyright: 'Copyright (C) 2019 Microsoft. All rights reserved',
@@ -52,14 +46,14 @@ export const config = {
darwinBundleDocumentType(["bowerrc"], 'resources/darwin/bower.icns'),
darwinBundleDocumentType(["c", "h"], 'resources/darwin/c.icns'),
darwinBundleDocumentType(["config", "editorconfig", "gitattributes", "gitconfig", "gitignore", "ini"], 'resources/darwin/config.icns'),
- darwinBundleDocumentType(["cc", "cpp", "cxx", "hh", "hpp", "hxx"], 'resources/darwin/cpp.icns'),
+ darwinBundleDocumentType(["cc", "cpp", "cxx", "c++", "hh", "hpp", "hxx", "h++"], 'resources/darwin/cpp.icns'),
darwinBundleDocumentType(["cs", "csx"], 'resources/darwin/csharp.icns'),
darwinBundleDocumentType(["css"], 'resources/darwin/css.icns'),
darwinBundleDocumentType(["go"], 'resources/darwin/go.icns'),
darwinBundleDocumentType(["asp", "aspx", "cshtml", "htm", "html", "jshtm", "jsp", "phtml", "shtml"], 'resources/darwin/html.icns'),
darwinBundleDocumentType(["jade"], 'resources/darwin/jade.icns'),
darwinBundleDocumentType(["jav", "java"], 'resources/darwin/java.icns'),
- darwinBundleDocumentType(["js", "jscsrc", "jshintrc", "mjs"], 'resources/darwin/javascript.icns'),
+ darwinBundleDocumentType(["js", "jscsrc", "jshintrc", "mjs", "cjs"], 'resources/darwin/javascript.icns'),
darwinBundleDocumentType(["json"], 'resources/darwin/json.icns'),
darwinBundleDocumentType(["less"], 'resources/darwin/less.icns'),
darwinBundleDocumentType(["markdown", "md", "mdoc", "mdown", "mdtext", "mdtxt", "mdwn", "mkd", "mkdn"], 'resources/darwin/markdown.icns'),
@@ -75,7 +69,7 @@ export const config = {
darwinBundleDocumentType(["vue"], 'resources/darwin/vue.icns'),
darwinBundleDocumentType(["ascx", "csproj", "dtd", "wxi", "wxl", "wxs", "xml", "xaml"], 'resources/darwin/xml.icns'),
darwinBundleDocumentType(["eyaml", "eyml", "yaml", "yml"], 'resources/darwin/yaml.icns'),
- darwinBundleDocumentType(["clj", "cljs", "cljx", "clojure", "code-workspace", "coffee", "ctp", "dockerfile", "dot", "edn", "fs", "fsi", "fsscript", "fsx", "handlebars", "hbs", "lua", "m", "makefile", "ml", "mli", "pl", "pl6", "pm", "pm6", "pod", "pp", "properties", "psgi", "pug", "r", "rs", "rt", "svg", "svgz", "t", "txt", "vb", "xcodeproj", "xcworkspace"], 'resources/darwin/default.icns')
+ darwinBundleDocumentType(["clj", "cljs", "cljx", "clojure", "code-workspace", "coffee", "containerfile", "ctp", "dockerfile", "dot", "edn", "fs", "fsi", "fsscript", "fsx", "handlebars", "hbs", "lua", "m", "makefile", "ml", "mli", "pl", "pl6", "pm", "pm6", "pod", "pp", "properties", "psgi", "pug", "r", "rs", "rt", "svg", "svgz", "t", "txt", "vb", "xcodeproj", "xcworkspace"], 'resources/darwin/default.icns')
],
darwinBundleURLTypes: [{
role: 'Viewer',
@@ -94,7 +88,7 @@ function getElectron(arch: string): () => NodeJS.ReadWriteStream {
return () => {
const electronOpts = _.extend({}, config, {
platform: process.platform,
- arch,
+ arch: arch === 'armhf' ? 'arm' : arch,
ffmpegChromium: true,
keepDefaultApp: true
});
@@ -108,7 +102,7 @@ function getElectron(arch: string): () => NodeJS.ReadWriteStream {
}
async function main(arch = process.arch): Promise {
- const version = getElectronVersion();
+ const version = util.getElectronVersion();
const electronPath = path.join(root, '.build', 'electron');
const versionFile = path.join(electronPath, 'version');
const isUpToDate = fs.existsSync(versionFile) && fs.readFileSync(versionFile, 'utf8') === `${version}`;
diff --git a/build/lib/eslint/code-import-patterns.js b/build/lib/eslint/code-import-patterns.js
new file mode 100644
index 0000000000000..0d508d1d00e37
--- /dev/null
+++ b/build/lib/eslint/code-import-patterns.js
@@ -0,0 +1,59 @@
+"use strict";
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+const path_1 = require("path");
+const minimatch = require("minimatch");
+const utils_1 = require("./utils");
+module.exports = new class {
+ constructor() {
+ this.meta = {
+ messages: {
+ badImport: 'Imports violates \'{{restrictions}}\' restrictions. See https://github.com/microsoft/vscode/wiki/Source-Code-Organization'
+ },
+ docs: {
+ url: 'https://github.com/microsoft/vscode/wiki/Source-Code-Organization'
+ }
+ };
+ }
+ create(context) {
+ const configs = context.options;
+ for (const config of configs) {
+ if (minimatch(context.getFilename(), config.target)) {
+ return utils_1.createImportRuleListener((node, value) => this._checkImport(context, config, node, value));
+ }
+ }
+ return {};
+ }
+ _checkImport(context, config, node, path) {
+ // resolve relative paths
+ if (path[0] === '.') {
+ path = path_1.join(context.getFilename(), path);
+ }
+ let restrictions;
+ if (typeof config.restrictions === 'string') {
+ restrictions = [config.restrictions];
+ }
+ else {
+ restrictions = config.restrictions;
+ }
+ let matched = false;
+ for (const pattern of restrictions) {
+ if (minimatch(path, pattern)) {
+ matched = true;
+ break;
+ }
+ }
+ if (!matched) {
+ // None of the restrictions matched
+ context.report({
+ loc: node.loc,
+ messageId: 'badImport',
+ data: {
+ restrictions: restrictions.join(' or ')
+ }
+ });
+ }
+ }
+};
diff --git a/build/lib/eslint/code-import-patterns.ts b/build/lib/eslint/code-import-patterns.ts
new file mode 100644
index 0000000000000..c3daadbf59a0d
--- /dev/null
+++ b/build/lib/eslint/code-import-patterns.ts
@@ -0,0 +1,75 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import * as eslint from 'eslint';
+import { TSESTree } from '@typescript-eslint/experimental-utils';
+import { join } from 'path';
+import * as minimatch from 'minimatch';
+import { createImportRuleListener } from './utils';
+
+interface ImportPatternsConfig {
+ target: string;
+ restrictions: string | string[];
+}
+
+export = new class implements eslint.Rule.RuleModule {
+
+ readonly meta: eslint.Rule.RuleMetaData = {
+ messages: {
+ badImport: 'Imports violates \'{{restrictions}}\' restrictions. See https://github.com/microsoft/vscode/wiki/Source-Code-Organization'
+ },
+ docs: {
+ url: 'https://github.com/microsoft/vscode/wiki/Source-Code-Organization'
+ }
+ };
+
+ create(context: eslint.Rule.RuleContext): eslint.Rule.RuleListener {
+
+ const configs = context.options;
+
+ for (const config of configs) {
+ if (minimatch(context.getFilename(), config.target)) {
+ return createImportRuleListener((node, value) => this._checkImport(context, config, node, value));
+ }
+ }
+
+ return {};
+ }
+
+ private _checkImport(context: eslint.Rule.RuleContext, config: ImportPatternsConfig, node: TSESTree.Node, path: string) {
+
+ // resolve relative paths
+ if (path[0] === '.') {
+ path = join(context.getFilename(), path);
+ }
+
+ let restrictions: string[];
+ if (typeof config.restrictions === 'string') {
+ restrictions = [config.restrictions];
+ } else {
+ restrictions = config.restrictions;
+ }
+
+ let matched = false;
+ for (const pattern of restrictions) {
+ if (minimatch(path, pattern)) {
+ matched = true;
+ break;
+ }
+ }
+
+ if (!matched) {
+ // None of the restrictions matched
+ context.report({
+ loc: node.loc,
+ messageId: 'badImport',
+ data: {
+ restrictions: restrictions.join(' or ')
+ }
+ });
+ }
+ }
+};
+
diff --git a/build/lib/eslint/code-layering.js b/build/lib/eslint/code-layering.js
new file mode 100644
index 0000000000000..db591f789c72c
--- /dev/null
+++ b/build/lib/eslint/code-layering.js
@@ -0,0 +1,68 @@
+"use strict";
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+const path_1 = require("path");
+const utils_1 = require("./utils");
+module.exports = new class {
+ constructor() {
+ this.meta = {
+ messages: {
+ layerbreaker: 'Bad layering. You are not allowed to access {{from}} from here, allowed layers are: [{{allowed}}]'
+ },
+ docs: {
+ url: 'https://github.com/microsoft/vscode/wiki/Source-Code-Organization'
+ }
+ };
+ }
+ create(context) {
+ const fileDirname = path_1.dirname(context.getFilename());
+ const parts = fileDirname.split(/\\|\//);
+ const ruleArgs = context.options[0];
+ let config;
+ for (let i = parts.length - 1; i >= 0; i--) {
+ if (ruleArgs[parts[i]]) {
+ config = {
+ allowed: new Set(ruleArgs[parts[i]]).add(parts[i]),
+ disallowed: new Set()
+ };
+ Object.keys(ruleArgs).forEach(key => {
+ if (!config.allowed.has(key)) {
+ config.disallowed.add(key);
+ }
+ });
+ break;
+ }
+ }
+ if (!config) {
+ // nothing
+ return {};
+ }
+ return utils_1.createImportRuleListener((node, path) => {
+ if (path[0] === '.') {
+ path = path_1.join(path_1.dirname(context.getFilename()), path);
+ }
+ const parts = path_1.dirname(path).split(/\\|\//);
+ for (let i = parts.length - 1; i >= 0; i--) {
+ const part = parts[i];
+ if (config.allowed.has(part)) {
+ // GOOD - same layer
+ break;
+ }
+ if (config.disallowed.has(part)) {
+ // BAD - wrong layer
+ context.report({
+ loc: node.loc,
+ messageId: 'layerbreaker',
+ data: {
+ from: part,
+ allowed: [...config.allowed.keys()].join(', ')
+ }
+ });
+ break;
+ }
+ }
+ });
+ }
+};
diff --git a/build/lib/eslint/code-layering.ts b/build/lib/eslint/code-layering.ts
new file mode 100644
index 0000000000000..cca72eeec71e4
--- /dev/null
+++ b/build/lib/eslint/code-layering.ts
@@ -0,0 +1,83 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import * as eslint from 'eslint';
+import { join, dirname } from 'path';
+import { createImportRuleListener } from './utils';
+
+type Config = {
+ allowed: Set;
+ disallowed: Set;
+};
+
+export = new class implements eslint.Rule.RuleModule {
+
+ readonly meta: eslint.Rule.RuleMetaData = {
+ messages: {
+ layerbreaker: 'Bad layering. You are not allowed to access {{from}} from here, allowed layers are: [{{allowed}}]'
+ },
+ docs: {
+ url: 'https://github.com/microsoft/vscode/wiki/Source-Code-Organization'
+ }
+ };
+
+ create(context: eslint.Rule.RuleContext): eslint.Rule.RuleListener {
+
+ const fileDirname = dirname(context.getFilename());
+ const parts = fileDirname.split(/\\|\//);
+ const ruleArgs = >context.options[0];
+
+ let config: Config | undefined;
+ for (let i = parts.length - 1; i >= 0; i--) {
+ if (ruleArgs[parts[i]]) {
+ config = {
+ allowed: new Set(ruleArgs[parts[i]]).add(parts[i]),
+ disallowed: new Set()
+ };
+ Object.keys(ruleArgs).forEach(key => {
+ if (!config!.allowed.has(key)) {
+ config!.disallowed.add(key);
+ }
+ });
+ break;
+ }
+ }
+
+ if (!config) {
+ // nothing
+ return {};
+ }
+
+ return createImportRuleListener((node, path) => {
+ if (path[0] === '.') {
+ path = join(dirname(context.getFilename()), path);
+ }
+
+ const parts = dirname(path).split(/\\|\//);
+ for (let i = parts.length - 1; i >= 0; i--) {
+ const part = parts[i];
+
+ if (config!.allowed.has(part)) {
+ // GOOD - same layer
+ break;
+ }
+
+ if (config!.disallowed.has(part)) {
+ // BAD - wrong layer
+ context.report({
+ loc: node.loc,
+ messageId: 'layerbreaker',
+ data: {
+ from: part,
+ allowed: [...config!.allowed.keys()].join(', ')
+ }
+ });
+ break;
+ }
+ }
+ });
+ }
+};
+
diff --git a/build/lib/eslint/code-no-nls-in-standalone-editor.js b/build/lib/eslint/code-no-nls-in-standalone-editor.js
new file mode 100644
index 0000000000000..d8955507bedc9
--- /dev/null
+++ b/build/lib/eslint/code-no-nls-in-standalone-editor.js
@@ -0,0 +1,38 @@
+"use strict";
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+const path_1 = require("path");
+const utils_1 = require("./utils");
+module.exports = new class NoNlsInStandaloneEditorRule {
+ constructor() {
+ this.meta = {
+ messages: {
+ noNls: 'Not allowed to import vs/nls in standalone editor modules. Use standaloneStrings.ts'
+ }
+ };
+ }
+ create(context) {
+ const fileName = context.getFilename();
+ if (/vs(\/|\\)editor(\/|\\)standalone(\/|\\)/.test(fileName)
+ || /vs(\/|\\)editor(\/|\\)common(\/|\\)standalone(\/|\\)/.test(fileName)
+ || /vs(\/|\\)editor(\/|\\)editor.api/.test(fileName)
+ || /vs(\/|\\)editor(\/|\\)editor.main/.test(fileName)
+ || /vs(\/|\\)editor(\/|\\)editor.worker/.test(fileName)) {
+ return utils_1.createImportRuleListener((node, path) => {
+ // resolve relative paths
+ if (path[0] === '.') {
+ path = path_1.join(context.getFilename(), path);
+ }
+ if (/vs(\/|\\)nls/.test(path)) {
+ context.report({
+ loc: node.loc,
+ messageId: 'noNls'
+ });
+ }
+ });
+ }
+ return {};
+ }
+};
diff --git a/build/lib/eslint/code-no-nls-in-standalone-editor.ts b/build/lib/eslint/code-no-nls-in-standalone-editor.ts
new file mode 100644
index 0000000000000..90c80dee70c9d
--- /dev/null
+++ b/build/lib/eslint/code-no-nls-in-standalone-editor.ts
@@ -0,0 +1,48 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import * as eslint from 'eslint';
+import { join } from 'path';
+import { createImportRuleListener } from './utils';
+
+export = new class NoNlsInStandaloneEditorRule implements eslint.Rule.RuleModule {
+
+ readonly meta: eslint.Rule.RuleMetaData = {
+ messages: {
+ noNls: 'Not allowed to import vs/nls in standalone editor modules. Use standaloneStrings.ts'
+ }
+ };
+
+ create(context: eslint.Rule.RuleContext): eslint.Rule.RuleListener {
+
+ const fileName = context.getFilename();
+ if (
+ /vs(\/|\\)editor(\/|\\)standalone(\/|\\)/.test(fileName)
+ || /vs(\/|\\)editor(\/|\\)common(\/|\\)standalone(\/|\\)/.test(fileName)
+ || /vs(\/|\\)editor(\/|\\)editor.api/.test(fileName)
+ || /vs(\/|\\)editor(\/|\\)editor.main/.test(fileName)
+ || /vs(\/|\\)editor(\/|\\)editor.worker/.test(fileName)
+ ) {
+ return createImportRuleListener((node, path) => {
+ // resolve relative paths
+ if (path[0] === '.') {
+ path = join(context.getFilename(), path);
+ }
+
+ if (
+ /vs(\/|\\)nls/.test(path)
+ ) {
+ context.report({
+ loc: node.loc,
+ messageId: 'noNls'
+ });
+ }
+ });
+ }
+
+ return {};
+ }
+};
+
diff --git a/build/lib/eslint/code-no-standalone-editor.js b/build/lib/eslint/code-no-standalone-editor.js
new file mode 100644
index 0000000000000..d9d6bb55b872f
--- /dev/null
+++ b/build/lib/eslint/code-no-standalone-editor.js
@@ -0,0 +1,41 @@
+"use strict";
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+const path_1 = require("path");
+const utils_1 = require("./utils");
+module.exports = new class NoNlsInStandaloneEditorRule {
+ constructor() {
+ this.meta = {
+ messages: {
+ badImport: 'Not allowed to import standalone editor modules.'
+ },
+ docs: {
+ url: 'https://github.com/microsoft/vscode/wiki/Source-Code-Organization'
+ }
+ };
+ }
+ create(context) {
+ if (/vs(\/|\\)editor/.test(context.getFilename())) {
+ // the vs/editor folder is allowed to use the standalone editor
+ return {};
+ }
+ return utils_1.createImportRuleListener((node, path) => {
+ // resolve relative paths
+ if (path[0] === '.') {
+ path = path_1.join(context.getFilename(), path);
+ }
+ if (/vs(\/|\\)editor(\/|\\)standalone(\/|\\)/.test(path)
+ || /vs(\/|\\)editor(\/|\\)common(\/|\\)standalone(\/|\\)/.test(path)
+ || /vs(\/|\\)editor(\/|\\)editor.api/.test(path)
+ || /vs(\/|\\)editor(\/|\\)editor.main/.test(path)
+ || /vs(\/|\\)editor(\/|\\)editor.worker/.test(path)) {
+ context.report({
+ loc: node.loc,
+ messageId: 'badImport'
+ });
+ }
+ });
+ }
+};
diff --git a/build/lib/eslint/code-no-standalone-editor.ts b/build/lib/eslint/code-no-standalone-editor.ts
new file mode 100644
index 0000000000000..898886d17d203
--- /dev/null
+++ b/build/lib/eslint/code-no-standalone-editor.ts
@@ -0,0 +1,50 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import * as eslint from 'eslint';
+import { join } from 'path';
+import { createImportRuleListener } from './utils';
+
+export = new class NoNlsInStandaloneEditorRule implements eslint.Rule.RuleModule {
+
+ readonly meta: eslint.Rule.RuleMetaData = {
+ messages: {
+ badImport: 'Not allowed to import standalone editor modules.'
+ },
+ docs: {
+ url: 'https://github.com/microsoft/vscode/wiki/Source-Code-Organization'
+ }
+ };
+
+ create(context: eslint.Rule.RuleContext): eslint.Rule.RuleListener {
+
+ if (/vs(\/|\\)editor/.test(context.getFilename())) {
+ // the vs/editor folder is allowed to use the standalone editor
+ return {};
+ }
+
+ return createImportRuleListener((node, path) => {
+
+ // resolve relative paths
+ if (path[0] === '.') {
+ path = join(context.getFilename(), path);
+ }
+
+ if (
+ /vs(\/|\\)editor(\/|\\)standalone(\/|\\)/.test(path)
+ || /vs(\/|\\)editor(\/|\\)common(\/|\\)standalone(\/|\\)/.test(path)
+ || /vs(\/|\\)editor(\/|\\)editor.api/.test(path)
+ || /vs(\/|\\)editor(\/|\\)editor.main/.test(path)
+ || /vs(\/|\\)editor(\/|\\)editor.worker/.test(path)
+ ) {
+ context.report({
+ loc: node.loc,
+ messageId: 'badImport'
+ });
+ }
+ });
+ }
+};
+
diff --git a/build/lib/eslint/code-no-unexternalized-strings.js b/build/lib/eslint/code-no-unexternalized-strings.js
new file mode 100644
index 0000000000000..28fce5b9a182b
--- /dev/null
+++ b/build/lib/eslint/code-no-unexternalized-strings.js
@@ -0,0 +1,111 @@
+"use strict";
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+var _a;
+const experimental_utils_1 = require("@typescript-eslint/experimental-utils");
+function isStringLiteral(node) {
+ return !!node && node.type === experimental_utils_1.AST_NODE_TYPES.Literal && typeof node.value === 'string';
+}
+function isDoubleQuoted(node) {
+ return node.raw[0] === '"' && node.raw[node.raw.length - 1] === '"';
+}
+module.exports = new (_a = class NoUnexternalizedStrings {
+ constructor() {
+ this.meta = {
+ messages: {
+ doubleQuoted: 'Only use double-quoted strings for externalized strings.',
+ badKey: 'The key \'{{key}}\' doesn\'t conform to a valid localize identifier.',
+ duplicateKey: 'Duplicate key \'{{key}}\' with different message value.',
+ badMessage: 'Message argument to \'{{message}}\' must be a string literal.'
+ }
+ };
+ }
+ create(context) {
+ const externalizedStringLiterals = new Map();
+ const doubleQuotedStringLiterals = new Set();
+ function collectDoubleQuotedStrings(node) {
+ if (isStringLiteral(node) && isDoubleQuoted(node)) {
+ doubleQuotedStringLiterals.add(node);
+ }
+ }
+ function visitLocalizeCall(node) {
+ // localize(key, message)
+ const [keyNode, messageNode] = node.arguments;
+ // (1)
+ // extract key so that it can be checked later
+ let key;
+ if (isStringLiteral(keyNode)) {
+ doubleQuotedStringLiterals.delete(keyNode); //todo@joh reconsider
+ key = keyNode.value;
+ }
+ else if (keyNode.type === experimental_utils_1.AST_NODE_TYPES.ObjectExpression) {
+ for (let property of keyNode.properties) {
+ if (property.type === experimental_utils_1.AST_NODE_TYPES.Property && !property.computed) {
+ if (property.key.type === experimental_utils_1.AST_NODE_TYPES.Identifier && property.key.name === 'key') {
+ if (isStringLiteral(property.value)) {
+ doubleQuotedStringLiterals.delete(property.value); //todo@joh reconsider
+ key = property.value.value;
+ break;
+ }
+ }
+ }
+ }
+ }
+ if (typeof key === 'string') {
+ let array = externalizedStringLiterals.get(key);
+ if (!array) {
+ array = [];
+ externalizedStringLiterals.set(key, array);
+ }
+ array.push({ call: node, message: messageNode });
+ }
+ // (2)
+ // remove message-argument from doubleQuoted list and make
+ // sure it is a string-literal
+ doubleQuotedStringLiterals.delete(messageNode);
+ if (!isStringLiteral(messageNode)) {
+ context.report({
+ loc: messageNode.loc,
+ messageId: 'badMessage',
+ data: { message: context.getSourceCode().getText(node) }
+ });
+ }
+ }
+ function reportBadStringsAndBadKeys() {
+ // (1)
+ // report all strings that are in double quotes
+ for (const node of doubleQuotedStringLiterals) {
+ context.report({ loc: node.loc, messageId: 'doubleQuoted' });
+ }
+ for (const [key, values] of externalizedStringLiterals) {
+ // (2)
+ // report all invalid NLS keys
+ if (!key.match(NoUnexternalizedStrings._rNlsKeys)) {
+ for (let value of values) {
+ context.report({ loc: value.call.loc, messageId: 'badKey', data: { key } });
+ }
+ }
+ // (2)
+ // report all invalid duplicates (same key, different message)
+ if (values.length > 1) {
+ for (let i = 1; i < values.length; i++) {
+ if (context.getSourceCode().getText(values[i - 1].message) !== context.getSourceCode().getText(values[i].message)) {
+ context.report({ loc: values[i].call.loc, messageId: 'duplicateKey', data: { key } });
+ }
+ }
+ }
+ }
+ }
+ return {
+ ['Literal']: (node) => collectDoubleQuotedStrings(node),
+ ['ExpressionStatement[directive] Literal:exit']: (node) => doubleQuotedStringLiterals.delete(node),
+ ['CallExpression[callee.type="MemberExpression"][callee.object.name="nls"][callee.property.name="localize"]:exit']: (node) => visitLocalizeCall(node),
+ ['CallExpression[callee.name="localize"][arguments.length>=2]:exit']: (node) => visitLocalizeCall(node),
+ ['Program:exit']: reportBadStringsAndBadKeys,
+ };
+ }
+ },
+ _a._rNlsKeys = /^[_a-zA-Z0-9][ .\-_a-zA-Z0-9]*$/,
+ _a);
diff --git a/build/lib/eslint/code-no-unexternalized-strings.ts b/build/lib/eslint/code-no-unexternalized-strings.ts
new file mode 100644
index 0000000000000..29db884cd9f31
--- /dev/null
+++ b/build/lib/eslint/code-no-unexternalized-strings.ts
@@ -0,0 +1,126 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import * as eslint from 'eslint';
+import { TSESTree, AST_NODE_TYPES } from '@typescript-eslint/experimental-utils';
+
+function isStringLiteral(node: TSESTree.Node | null | undefined): node is TSESTree.StringLiteral {
+ return !!node && node.type === AST_NODE_TYPES.Literal && typeof node.value === 'string';
+}
+
+function isDoubleQuoted(node: TSESTree.StringLiteral): boolean {
+ return node.raw[0] === '"' && node.raw[node.raw.length - 1] === '"';
+}
+
+export = new class NoUnexternalizedStrings implements eslint.Rule.RuleModule {
+
+ private static _rNlsKeys = /^[_a-zA-Z0-9][ .\-_a-zA-Z0-9]*$/;
+
+ readonly meta: eslint.Rule.RuleMetaData = {
+ messages: {
+ doubleQuoted: 'Only use double-quoted strings for externalized strings.',
+ badKey: 'The key \'{{key}}\' doesn\'t conform to a valid localize identifier.',
+ duplicateKey: 'Duplicate key \'{{key}}\' with different message value.',
+ badMessage: 'Message argument to \'{{message}}\' must be a string literal.'
+ }
+ };
+
+ create(context: eslint.Rule.RuleContext): eslint.Rule.RuleListener {
+
+ const externalizedStringLiterals = new Map();
+ const doubleQuotedStringLiterals = new Set();
+
+ function collectDoubleQuotedStrings(node: TSESTree.Literal) {
+ if (isStringLiteral(node) && isDoubleQuoted(node)) {
+ doubleQuotedStringLiterals.add(node);
+ }
+ }
+
+ function visitLocalizeCall(node: TSESTree.CallExpression) {
+
+ // localize(key, message)
+ const [keyNode, messageNode] = (node).arguments;
+
+ // (1)
+ // extract key so that it can be checked later
+ let key: string | undefined;
+ if (isStringLiteral(keyNode)) {
+ doubleQuotedStringLiterals.delete(keyNode); //todo@joh reconsider
+ key = keyNode.value;
+
+ } else if (keyNode.type === AST_NODE_TYPES.ObjectExpression) {
+ for (let property of keyNode.properties) {
+ if (property.type === AST_NODE_TYPES.Property && !property.computed) {
+ if (property.key.type === AST_NODE_TYPES.Identifier && property.key.name === 'key') {
+ if (isStringLiteral(property.value)) {
+ doubleQuotedStringLiterals.delete(property.value); //todo@joh reconsider
+ key = property.value.value;
+ break;
+ }
+ }
+ }
+ }
+ }
+ if (typeof key === 'string') {
+ let array = externalizedStringLiterals.get(key);
+ if (!array) {
+ array = [];
+ externalizedStringLiterals.set(key, array);
+ }
+ array.push({ call: node, message: messageNode });
+ }
+
+ // (2)
+ // remove message-argument from doubleQuoted list and make
+ // sure it is a string-literal
+ doubleQuotedStringLiterals.delete(messageNode);
+ if (!isStringLiteral(messageNode)) {
+ context.report({
+ loc: messageNode.loc,
+ messageId: 'badMessage',
+ data: { message: context.getSourceCode().getText(node) }
+ });
+ }
+ }
+
+ function reportBadStringsAndBadKeys() {
+ // (1)
+ // report all strings that are in double quotes
+ for (const node of doubleQuotedStringLiterals) {
+ context.report({ loc: node.loc, messageId: 'doubleQuoted' });
+ }
+
+ for (const [key, values] of externalizedStringLiterals) {
+
+ // (2)
+ // report all invalid NLS keys
+ if (!key.match(NoUnexternalizedStrings._rNlsKeys)) {
+ for (let value of values) {
+ context.report({ loc: value.call.loc, messageId: 'badKey', data: { key } });
+ }
+ }
+
+ // (2)
+ // report all invalid duplicates (same key, different message)
+ if (values.length > 1) {
+ for (let i = 1; i < values.length; i++) {
+ if (context.getSourceCode().getText(values[i - 1].message) !== context.getSourceCode().getText(values[i].message)) {
+ context.report({ loc: values[i].call.loc, messageId: 'duplicateKey', data: { key } });
+ }
+ }
+ }
+ }
+ }
+
+ return {
+ ['Literal']: (node: any) => collectDoubleQuotedStrings(node),
+ ['ExpressionStatement[directive] Literal:exit']: (node: any) => doubleQuotedStringLiterals.delete(node),
+ ['CallExpression[callee.type="MemberExpression"][callee.object.name="nls"][callee.property.name="localize"]:exit']: (node: any) => visitLocalizeCall(node),
+ ['CallExpression[callee.name="localize"][arguments.length>=2]:exit']: (node: any) => visitLocalizeCall(node),
+ ['Program:exit']: reportBadStringsAndBadKeys,
+ };
+ }
+};
+
diff --git a/build/lib/eslint/code-no-unused-expressions.js b/build/lib/eslint/code-no-unused-expressions.js
new file mode 100644
index 0000000000000..c7b175513117c
--- /dev/null
+++ b/build/lib/eslint/code-no-unused-expressions.js
@@ -0,0 +1,145 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+// FORKED FROM https://github.com/eslint/eslint/blob/b23ad0d789a909baf8d7c41a35bc53df932eaf30/lib/rules/no-unused-expressions.js
+// and added support for `OptionalCallExpression`, see https://github.com/facebook/create-react-app/issues/8107 and https://github.com/eslint/eslint/issues/12642
+
+/**
+ * @fileoverview Flag expressions in statement position that do not side effect
+ * @author Michael Ficarra
+ */
+
+'use strict';
+
+//------------------------------------------------------------------------------
+// Rule Definition
+//------------------------------------------------------------------------------
+
+module.exports = {
+ meta: {
+ type: 'suggestion',
+
+ docs: {
+ description: 'disallow unused expressions',
+ category: 'Best Practices',
+ recommended: false,
+ url: 'https://eslint.org/docs/rules/no-unused-expressions'
+ },
+
+ schema: [
+ {
+ type: 'object',
+ properties: {
+ allowShortCircuit: {
+ type: 'boolean',
+ default: false
+ },
+ allowTernary: {
+ type: 'boolean',
+ default: false
+ },
+ allowTaggedTemplates: {
+ type: 'boolean',
+ default: false
+ }
+ },
+ additionalProperties: false
+ }
+ ]
+ },
+
+ create(context) {
+ const config = context.options[0] || {},
+ allowShortCircuit = config.allowShortCircuit || false,
+ allowTernary = config.allowTernary || false,
+ allowTaggedTemplates = config.allowTaggedTemplates || false;
+
+ // eslint-disable-next-line jsdoc/require-description
+ /**
+ * @param {ASTNode} node any node
+ * @returns {boolean} whether the given node structurally represents a directive
+ */
+ function looksLikeDirective(node) {
+ return node.type === 'ExpressionStatement' &&
+ node.expression.type === 'Literal' && typeof node.expression.value === 'string';
+ }
+
+ // eslint-disable-next-line jsdoc/require-description
+ /**
+ * @param {Function} predicate ([a] -> Boolean) the function used to make the determination
+ * @param {a[]} list the input list
+ * @returns {a[]} the leading sequence of members in the given list that pass the given predicate
+ */
+ function takeWhile(predicate, list) {
+ for (let i = 0; i < list.length; ++i) {
+ if (!predicate(list[i])) {
+ return list.slice(0, i);
+ }
+ }
+ return list.slice();
+ }
+
+ // eslint-disable-next-line jsdoc/require-description
+ /**
+ * @param {ASTNode} node a Program or BlockStatement node
+ * @returns {ASTNode[]} the leading sequence of directive nodes in the given node's body
+ */
+ function directives(node) {
+ return takeWhile(looksLikeDirective, node.body);
+ }
+
+ // eslint-disable-next-line jsdoc/require-description
+ /**
+ * @param {ASTNode} node any node
+ * @param {ASTNode[]} ancestors the given node's ancestors
+ * @returns {boolean} whether the given node is considered a directive in its current position
+ */
+ function isDirective(node, ancestors) {
+ const parent = ancestors[ancestors.length - 1],
+ grandparent = ancestors[ancestors.length - 2];
+
+ return (parent.type === 'Program' || parent.type === 'BlockStatement' &&
+ (/Function/u.test(grandparent.type))) &&
+ directives(parent).indexOf(node) >= 0;
+ }
+
+ /**
+ * Determines whether or not a given node is a valid expression. Recurses on short circuit eval and ternary nodes if enabled by flags.
+ * @param {ASTNode} node any node
+ * @returns {boolean} whether the given node is a valid expression
+ */
+ function isValidExpression(node) {
+ if (allowTernary) {
+
+ // Recursive check for ternary and logical expressions
+ if (node.type === 'ConditionalExpression') {
+ return isValidExpression(node.consequent) && isValidExpression(node.alternate);
+ }
+ }
+
+ if (allowShortCircuit) {
+ if (node.type === 'LogicalExpression') {
+ return isValidExpression(node.right);
+ }
+ }
+
+ if (allowTaggedTemplates && node.type === 'TaggedTemplateExpression') {
+ return true;
+ }
+
+ return /^(?:Assignment|OptionalCall|Call|New|Update|Yield|Await)Expression$/u.test(node.type) ||
+ (node.type === 'UnaryExpression' && ['delete', 'void'].indexOf(node.operator) >= 0);
+ }
+
+ return {
+ ExpressionStatement(node) {
+ if (!isValidExpression(node.expression) && !isDirective(node, context.getAncestors())) {
+ context.report({ node, message: 'Expected an assignment or function call and instead saw an expression.' });
+ }
+ }
+ };
+
+ }
+};
diff --git a/build/lib/eslint/code-translation-remind.js b/build/lib/eslint/code-translation-remind.js
new file mode 100644
index 0000000000000..a276e7c0028aa
--- /dev/null
+++ b/build/lib/eslint/code-translation-remind.js
@@ -0,0 +1,57 @@
+"use strict";
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+var _a;
+const fs_1 = require("fs");
+const utils_1 = require("./utils");
+module.exports = new (_a = class TranslationRemind {
+ constructor() {
+ this.meta = {
+ messages: {
+ missing: 'Please add \'{{resource}}\' to ./build/lib/i18n.resources.json file to use translations here.'
+ }
+ };
+ }
+ create(context) {
+ return utils_1.createImportRuleListener((node, path) => this._checkImport(context, node, path));
+ }
+ _checkImport(context, node, path) {
+ if (path !== TranslationRemind.NLS_MODULE) {
+ return;
+ }
+ const currentFile = context.getFilename();
+ const matchService = currentFile.match(/vs\/workbench\/services\/\w+/);
+ const matchPart = currentFile.match(/vs\/workbench\/contrib\/\w+/);
+ if (!matchService && !matchPart) {
+ return;
+ }
+ const resource = matchService ? matchService[0] : matchPart[0];
+ let resourceDefined = false;
+ let json;
+ try {
+ json = fs_1.readFileSync('./build/lib/i18n.resources.json', 'utf8');
+ }
+ catch (e) {
+ console.error('[translation-remind rule]: File with resources to pull from Transifex was not found. Aborting translation resource check for newly defined workbench part/service.');
+ return;
+ }
+ const workbenchResources = JSON.parse(json).workbench;
+ workbenchResources.forEach((existingResource) => {
+ if (existingResource.name === resource) {
+ resourceDefined = true;
+ return;
+ }
+ });
+ if (!resourceDefined) {
+ context.report({
+ loc: node.loc,
+ messageId: 'missing',
+ data: { resource }
+ });
+ }
+ }
+ },
+ _a.NLS_MODULE = 'vs/nls',
+ _a);
diff --git a/build/lib/eslint/code-translation-remind.ts b/build/lib/eslint/code-translation-remind.ts
new file mode 100644
index 0000000000000..1ce01107a72a5
--- /dev/null
+++ b/build/lib/eslint/code-translation-remind.ts
@@ -0,0 +1,67 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import * as eslint from 'eslint';
+import { TSESTree } from '@typescript-eslint/experimental-utils';
+import { readFileSync } from 'fs';
+import { createImportRuleListener } from './utils';
+
+
+export = new class TranslationRemind implements eslint.Rule.RuleModule {
+
+ private static NLS_MODULE = 'vs/nls';
+
+ readonly meta: eslint.Rule.RuleMetaData = {
+ messages: {
+ missing: 'Please add \'{{resource}}\' to ./build/lib/i18n.resources.json file to use translations here.'
+ }
+ };
+
+ create(context: eslint.Rule.RuleContext): eslint.Rule.RuleListener {
+ return createImportRuleListener((node, path) => this._checkImport(context, node, path));
+ }
+
+ private _checkImport(context: eslint.Rule.RuleContext, node: TSESTree.Node, path: string) {
+
+ if (path !== TranslationRemind.NLS_MODULE) {
+ return;
+ }
+
+ const currentFile = context.getFilename();
+ const matchService = currentFile.match(/vs\/workbench\/services\/\w+/);
+ const matchPart = currentFile.match(/vs\/workbench\/contrib\/\w+/);
+ if (!matchService && !matchPart) {
+ return;
+ }
+
+ const resource = matchService ? matchService[0] : matchPart![0];
+ let resourceDefined = false;
+
+ let json;
+ try {
+ json = readFileSync('./build/lib/i18n.resources.json', 'utf8');
+ } catch (e) {
+ console.error('[translation-remind rule]: File with resources to pull from Transifex was not found. Aborting translation resource check for newly defined workbench part/service.');
+ return;
+ }
+ const workbenchResources = JSON.parse(json).workbench;
+
+ workbenchResources.forEach((existingResource: any) => {
+ if (existingResource.name === resource) {
+ resourceDefined = true;
+ return;
+ }
+ });
+
+ if (!resourceDefined) {
+ context.report({
+ loc: node.loc,
+ messageId: 'missing',
+ data: { resource }
+ });
+ }
+ }
+};
+
diff --git a/build/lib/eslint/utils.js b/build/lib/eslint/utils.js
new file mode 100644
index 0000000000000..c58e4e24be1e1
--- /dev/null
+++ b/build/lib/eslint/utils.js
@@ -0,0 +1,37 @@
+"use strict";
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.createImportRuleListener = void 0;
+function createImportRuleListener(validateImport) {
+ function _checkImport(node) {
+ if (node && node.type === 'Literal' && typeof node.value === 'string') {
+ validateImport(node, node.value);
+ }
+ }
+ return {
+ // import ??? from 'module'
+ ImportDeclaration: (node) => {
+ _checkImport(node.source);
+ },
+ // import('module').then(...) OR await import('module')
+ ['CallExpression[callee.type="Import"][arguments.length=1] > Literal']: (node) => {
+ _checkImport(node);
+ },
+ // import foo = ...
+ ['TSImportEqualsDeclaration > TSExternalModuleReference > Literal']: (node) => {
+ _checkImport(node);
+ },
+ // export ?? from 'module'
+ ExportAllDeclaration: (node) => {
+ _checkImport(node.source);
+ },
+ // export {foo} from 'module'
+ ExportNamedDeclaration: (node) => {
+ _checkImport(node.source);
+ },
+ };
+}
+exports.createImportRuleListener = createImportRuleListener;
diff --git a/build/lib/eslint/utils.ts b/build/lib/eslint/utils.ts
new file mode 100644
index 0000000000000..428832e9cf97a
--- /dev/null
+++ b/build/lib/eslint/utils.ts
@@ -0,0 +1,40 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import * as eslint from 'eslint';
+import { TSESTree } from '@typescript-eslint/experimental-utils';
+
+export function createImportRuleListener(validateImport: (node: TSESTree.Literal, value: string) => any): eslint.Rule.RuleListener {
+
+ function _checkImport(node: TSESTree.Node | null) {
+ if (node && node.type === 'Literal' && typeof node.value === 'string') {
+ validateImport(node, node.value);
+ }
+ }
+
+ return {
+ // import ??? from 'module'
+ ImportDeclaration: (node: any) => {
+ _checkImport((node).source);
+ },
+ // import('module').then(...) OR await import('module')
+ ['CallExpression[callee.type="Import"][arguments.length=1] > Literal']: (node: any) => {
+ _checkImport(node);
+ },
+ // import foo = ...
+ ['TSImportEqualsDeclaration > TSExternalModuleReference > Literal']: (node: any) => {
+ _checkImport(node);
+ },
+ // export ?? from 'module'
+ ExportAllDeclaration: (node: any) => {
+ _checkImport((node).source);
+ },
+ // export {foo} from 'module'
+ ExportNamedDeclaration: (node: any) => {
+ _checkImport((node).source);
+ },
+
+ };
+}
diff --git a/build/lib/eslint/vscode-dts-create-func.js b/build/lib/eslint/vscode-dts-create-func.js
new file mode 100644
index 0000000000000..5a27bf51c80f8
--- /dev/null
+++ b/build/lib/eslint/vscode-dts-create-func.js
@@ -0,0 +1,35 @@
+"use strict";
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+const experimental_utils_1 = require("@typescript-eslint/experimental-utils");
+module.exports = new class ApiLiteralOrTypes {
+ constructor() {
+ this.meta = {
+ docs: { url: 'https://github.com/microsoft/vscode/wiki/Extension-API-guidelines#creating-objects' },
+ messages: { sync: '`createXYZ`-functions are constructor-replacements and therefore must return sync', }
+ };
+ }
+ create(context) {
+ return {
+ ['TSDeclareFunction Identifier[name=/create.*/]']: (node) => {
+ var _a;
+ const decl = node.parent;
+ if (((_a = decl.returnType) === null || _a === void 0 ? void 0 : _a.typeAnnotation.type) !== experimental_utils_1.AST_NODE_TYPES.TSTypeReference) {
+ return;
+ }
+ if (decl.returnType.typeAnnotation.typeName.type !== experimental_utils_1.AST_NODE_TYPES.Identifier) {
+ return;
+ }
+ const ident = decl.returnType.typeAnnotation.typeName.name;
+ if (ident === 'Promise' || ident === 'Thenable') {
+ context.report({
+ node,
+ messageId: 'sync'
+ });
+ }
+ }
+ };
+ }
+};
diff --git a/build/lib/eslint/vscode-dts-create-func.ts b/build/lib/eslint/vscode-dts-create-func.ts
new file mode 100644
index 0000000000000..295d099da7ce4
--- /dev/null
+++ b/build/lib/eslint/vscode-dts-create-func.ts
@@ -0,0 +1,40 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import * as eslint from 'eslint';
+import { TSESTree, AST_NODE_TYPES } from '@typescript-eslint/experimental-utils';
+
+export = new class ApiLiteralOrTypes implements eslint.Rule.RuleModule {
+
+ readonly meta: eslint.Rule.RuleMetaData = {
+ docs: { url: 'https://github.com/microsoft/vscode/wiki/Extension-API-guidelines#creating-objects' },
+ messages: { sync: '`createXYZ`-functions are constructor-replacements and therefore must return sync', }
+ };
+
+ create(context: eslint.Rule.RuleContext): eslint.Rule.RuleListener {
+
+ return {
+ ['TSDeclareFunction Identifier[name=/create.*/]']: (node: any) => {
+
+ const decl = (node).parent;
+
+ if (decl.returnType?.typeAnnotation.type !== AST_NODE_TYPES.TSTypeReference) {
+ return;
+ }
+ if (decl.returnType.typeAnnotation.typeName.type !== AST_NODE_TYPES.Identifier) {
+ return;
+ }
+
+ const ident = decl.returnType.typeAnnotation.typeName.name;
+ if (ident === 'Promise' || ident === 'Thenable') {
+ context.report({
+ node,
+ messageId: 'sync'
+ });
+ }
+ }
+ };
+ }
+};
diff --git a/build/lib/eslint/vscode-dts-event-naming.js b/build/lib/eslint/vscode-dts-event-naming.js
new file mode 100644
index 0000000000000..388ccf2f80401
--- /dev/null
+++ b/build/lib/eslint/vscode-dts-event-naming.js
@@ -0,0 +1,87 @@
+"use strict";
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+var _a;
+const experimental_utils_1 = require("@typescript-eslint/experimental-utils");
+module.exports = new (_a = class ApiEventNaming {
+ constructor() {
+ this.meta = {
+ docs: {
+ url: 'https://github.com/microsoft/vscode/wiki/Extension-API-guidelines#event-naming'
+ },
+ messages: {
+ naming: 'Event names must follow this patten: `on[Did|Will]`',
+ verb: 'Unknown verb \'{{verb}}\' - is this really a verb? Iff so, then add this verb to the configuration',
+ subject: 'Unknown subject \'{{subject}}\' - This subject has not been used before but it should refer to something in the API',
+ unknown: 'UNKNOWN event declaration, lint-rule needs tweaking'
+ }
+ };
+ }
+ create(context) {
+ const config = context.options[0];
+ const allowed = new Set(config.allowed);
+ const verbs = new Set(config.verbs);
+ return {
+ ['TSTypeAnnotation TSTypeReference Identifier[name="Event"]']: (node) => {
+ var _a, _b;
+ const def = (_b = (_a = node.parent) === null || _a === void 0 ? void 0 : _a.parent) === null || _b === void 0 ? void 0 : _b.parent;
+ const ident = this.getIdent(def);
+ if (!ident) {
+ // event on unknown structure...
+ return context.report({
+ node,
+ message: 'unknown'
+ });
+ }
+ if (allowed.has(ident.name)) {
+ // configured exception
+ return;
+ }
+ const match = ApiEventNaming._nameRegExp.exec(ident.name);
+ if (!match) {
+ context.report({
+ node: ident,
+ messageId: 'naming'
+ });
+ return;
+ }
+ // check that is spelled out (configured) as verb
+ if (!verbs.has(match[2].toLowerCase())) {
+ context.report({
+ node: ident,
+ messageId: 'verb',
+ data: { verb: match[2] }
+ });
+ }
+ // check that a subject (if present) has occurred
+ if (match[3]) {
+ const regex = new RegExp(match[3], 'ig');
+ const parts = context.getSourceCode().getText().split(regex);
+ if (parts.length < 3) {
+ context.report({
+ node: ident,
+ messageId: 'subject',
+ data: { subject: match[3] }
+ });
+ }
+ }
+ }
+ };
+ }
+ getIdent(def) {
+ if (!def) {
+ return;
+ }
+ if (def.type === experimental_utils_1.AST_NODE_TYPES.Identifier) {
+ return def;
+ }
+ else if ((def.type === experimental_utils_1.AST_NODE_TYPES.TSPropertySignature || def.type === experimental_utils_1.AST_NODE_TYPES.ClassProperty) && def.key.type === experimental_utils_1.AST_NODE_TYPES.Identifier) {
+ return def.key;
+ }
+ return this.getIdent(def.parent);
+ }
+ },
+ _a._nameRegExp = /on(Did|Will)([A-Z][a-z]+)([A-Z][a-z]+)?/,
+ _a);
diff --git a/build/lib/eslint/vscode-dts-event-naming.ts b/build/lib/eslint/vscode-dts-event-naming.ts
new file mode 100644
index 0000000000000..5ed8818fe44b8
--- /dev/null
+++ b/build/lib/eslint/vscode-dts-event-naming.ts
@@ -0,0 +1,98 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import * as eslint from 'eslint';
+import { TSESTree, AST_NODE_TYPES } from '@typescript-eslint/experimental-utils';
+
+export = new class ApiEventNaming implements eslint.Rule.RuleModule {
+
+ private static _nameRegExp = /on(Did|Will)([A-Z][a-z]+)([A-Z][a-z]+)?/;
+
+ readonly meta: eslint.Rule.RuleMetaData = {
+ docs: {
+ url: 'https://github.com/microsoft/vscode/wiki/Extension-API-guidelines#event-naming'
+ },
+ messages: {
+ naming: 'Event names must follow this patten: `on[Did|Will]`',
+ verb: 'Unknown verb \'{{verb}}\' - is this really a verb? Iff so, then add this verb to the configuration',
+ subject: 'Unknown subject \'{{subject}}\' - This subject has not been used before but it should refer to something in the API',
+ unknown: 'UNKNOWN event declaration, lint-rule needs tweaking'
+ }
+ };
+
+ create(context: eslint.Rule.RuleContext): eslint.Rule.RuleListener {
+
+ const config = <{ allowed: string[], verbs: string[] }>context.options[0];
+ const allowed = new Set(config.allowed);
+ const verbs = new Set(config.verbs);
+
+ return {
+ ['TSTypeAnnotation TSTypeReference Identifier[name="Event"]']: (node: any) => {
+
+ const def = (node).parent?.parent?.parent;
+ const ident = this.getIdent(def);
+
+ if (!ident) {
+ // event on unknown structure...
+ return context.report({
+ node,
+ message: 'unknown'
+ });
+ }
+
+ if (allowed.has(ident.name)) {
+ // configured exception
+ return;
+ }
+
+ const match = ApiEventNaming._nameRegExp.exec(ident.name);
+ if (!match) {
+ context.report({
+ node: ident,
+ messageId: 'naming'
+ });
+ return;
+ }
+
+ // check that is spelled out (configured) as verb
+ if (!verbs.has(match[2].toLowerCase())) {
+ context.report({
+ node: ident,
+ messageId: 'verb',
+ data: { verb: match[2] }
+ });
+ }
+
+ // check that a subject (if present) has occurred
+ if (match[3]) {
+ const regex = new RegExp(match[3], 'ig');
+ const parts = context.getSourceCode().getText().split(regex);
+ if (parts.length < 3) {
+ context.report({
+ node: ident,
+ messageId: 'subject',
+ data: { subject: match[3] }
+ });
+ }
+ }
+ }
+ };
+ }
+
+ private getIdent(def: TSESTree.Node | undefined): TSESTree.Identifier | undefined {
+ if (!def) {
+ return;
+ }
+
+ if (def.type === AST_NODE_TYPES.Identifier) {
+ return def;
+ } else if ((def.type === AST_NODE_TYPES.TSPropertySignature || def.type === AST_NODE_TYPES.ClassProperty) && def.key.type === AST_NODE_TYPES.Identifier) {
+ return def.key;
+ }
+
+ return this.getIdent(def.parent);
+ }
+};
+
diff --git a/build/lib/eslint/vscode-dts-interface-naming.js b/build/lib/eslint/vscode-dts-interface-naming.js
new file mode 100644
index 0000000000000..70ca810825ba0
--- /dev/null
+++ b/build/lib/eslint/vscode-dts-interface-naming.js
@@ -0,0 +1,30 @@
+"use strict";
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+var _a;
+module.exports = new (_a = class ApiInterfaceNaming {
+ constructor() {
+ this.meta = {
+ messages: {
+ naming: 'Interfaces must not be prefixed with uppercase `I`',
+ }
+ };
+ }
+ create(context) {
+ return {
+ ['TSInterfaceDeclaration Identifier']: (node) => {
+ const name = node.name;
+ if (ApiInterfaceNaming._nameRegExp.test(name)) {
+ context.report({
+ node,
+ messageId: 'naming'
+ });
+ }
+ }
+ };
+ }
+ },
+ _a._nameRegExp = /I[A-Z]/,
+ _a);
diff --git a/build/lib/eslint/vscode-dts-interface-naming.ts b/build/lib/eslint/vscode-dts-interface-naming.ts
new file mode 100644
index 0000000000000..d9ec4e8c34cbe
--- /dev/null
+++ b/build/lib/eslint/vscode-dts-interface-naming.ts
@@ -0,0 +1,35 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import * as eslint from 'eslint';
+import { TSESTree } from '@typescript-eslint/experimental-utils';
+
+export = new class ApiInterfaceNaming implements eslint.Rule.RuleModule {
+
+ private static _nameRegExp = /I[A-Z]/;
+
+ readonly meta: eslint.Rule.RuleMetaData = {
+ messages: {
+ naming: 'Interfaces must not be prefixed with uppercase `I`',
+ }
+ };
+
+ create(context: eslint.Rule.RuleContext): eslint.Rule.RuleListener {
+
+ return {
+ ['TSInterfaceDeclaration Identifier']: (node: any) => {
+
+ const name = (node).name;
+ if (ApiInterfaceNaming._nameRegExp.test(name)) {
+ context.report({
+ node,
+ messageId: 'naming'
+ });
+ }
+ }
+ };
+ }
+};
+
diff --git a/build/lib/eslint/vscode-dts-literal-or-types.js b/build/lib/eslint/vscode-dts-literal-or-types.js
new file mode 100644
index 0000000000000..e07dfc6de28fe
--- /dev/null
+++ b/build/lib/eslint/vscode-dts-literal-or-types.js
@@ -0,0 +1,27 @@
+"use strict";
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+module.exports = new class ApiLiteralOrTypes {
+ constructor() {
+ this.meta = {
+ docs: { url: 'https://github.com/microsoft/vscode/wiki/Extension-API-guidelines#enums' },
+ messages: { useEnum: 'Use enums, not literal-or-types', }
+ };
+ }
+ create(context) {
+ return {
+ ['TSTypeAnnotation TSUnionType TSLiteralType']: (node) => {
+ var _a;
+ if (((_a = node.literal) === null || _a === void 0 ? void 0 : _a.type) === 'TSNullKeyword') {
+ return;
+ }
+ context.report({
+ node: node,
+ messageId: 'useEnum'
+ });
+ }
+ };
+ }
+};
diff --git a/build/lib/eslint/vscode-dts-literal-or-types.ts b/build/lib/eslint/vscode-dts-literal-or-types.ts
new file mode 100644
index 0000000000000..fe4befd84e794
--- /dev/null
+++ b/build/lib/eslint/vscode-dts-literal-or-types.ts
@@ -0,0 +1,28 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import * as eslint from 'eslint';
+
+export = new class ApiLiteralOrTypes implements eslint.Rule.RuleModule {
+
+ readonly meta: eslint.Rule.RuleMetaData = {
+ docs: { url: 'https://github.com/microsoft/vscode/wiki/Extension-API-guidelines#enums' },
+ messages: { useEnum: 'Use enums, not literal-or-types', }
+ };
+
+ create(context: eslint.Rule.RuleContext): eslint.Rule.RuleListener {
+ return {
+ ['TSTypeAnnotation TSUnionType TSLiteralType']: (node: any) => {
+ if (node.literal?.type === 'TSNullKeyword') {
+ return;
+ }
+ context.report({
+ node: node,
+ messageId: 'useEnum'
+ });
+ }
+ };
+ }
+};
diff --git a/build/lib/extensions.js b/build/lib/extensions.js
index c4385655eb929..fe0deffc6d0c1 100644
--- a/build/lib/extensions.js
+++ b/build/lib/extensions.js
@@ -4,6 +4,7 @@
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
Object.defineProperty(exports, "__esModule", { value: true });
+exports.translatePackageJSON = exports.scanBuiltinExtensions = exports.packageMarketplaceExtensionsStream = exports.packageLocalExtensionsStream = exports.fromMarketplace = void 0;
const es = require("event-stream");
const fs = require("fs");
const glob = require("glob");
@@ -21,33 +22,66 @@ const fancyLog = require("fancy-log");
const ansiColors = require("ansi-colors");
const buffer = require('gulp-buffer');
const json = require("gulp-json-editor");
+const jsoncParser = require("jsonc-parser");
const webpack = require('webpack');
const webpackGulp = require('webpack-stream');
const util = require('./util');
const root = path.dirname(path.dirname(__dirname));
const commit = util.getVersion(root);
const sourceMappingURLBase = `https://ticino.blob.core.windows.net/sourcemaps/${commit}`;
-function fromLocal(extensionPath) {
- const webpackFilename = path.join(extensionPath, 'extension.webpack.config.js');
- const input = fs.existsSync(webpackFilename)
- ? fromLocalWebpack(extensionPath)
- : fromLocalNormal(extensionPath);
- const tmLanguageJsonFilter = filter('**/*.tmLanguage.json', { restore: true });
+function minifyExtensionResources(input) {
+ const jsonFilter = filter(['**/*.json', '**/*.code-snippets'], { restore: true });
+ return input
+ .pipe(jsonFilter)
+ .pipe(buffer())
+ .pipe(es.mapSync((f) => {
+ const errors = [];
+ const value = jsoncParser.parse(f.contents.toString('utf8'), errors);
+ if (errors.length === 0) {
+ // file parsed OK => just stringify to drop whitespace and comments
+ f.contents = Buffer.from(JSON.stringify(value));
+ }
+ return f;
+ }))
+ .pipe(jsonFilter.restore);
+}
+function updateExtensionPackageJSON(input, update) {
+ const packageJsonFilter = filter('extensions/*/package.json', { restore: true });
return input
- .pipe(tmLanguageJsonFilter)
+ .pipe(packageJsonFilter)
.pipe(buffer())
.pipe(es.mapSync((f) => {
- f.contents = Buffer.from(JSON.stringify(JSON.parse(f.contents.toString('utf8'))));
+ const data = JSON.parse(f.contents.toString('utf8'));
+ f.contents = Buffer.from(JSON.stringify(update(data)));
return f;
}))
- .pipe(tmLanguageJsonFilter.restore);
+ .pipe(packageJsonFilter.restore);
}
-function fromLocalWebpack(extensionPath) {
+function fromLocal(extensionPath, forWeb) {
+ const webpackConfigFileName = forWeb ? 'extension-browser.webpack.config.js' : 'extension.webpack.config.js';
+ const isWebPacked = fs.existsSync(path.join(extensionPath, webpackConfigFileName));
+ let input = isWebPacked
+ ? fromLocalWebpack(extensionPath, webpackConfigFileName)
+ : fromLocalNormal(extensionPath);
+ if (isWebPacked) {
+ input = updateExtensionPackageJSON(input, (data) => {
+ delete data.scripts;
+ delete data.dependencies;
+ delete data.devDependencies;
+ if (data.main) {
+ data.main = data.main.replace('/out/', /dist/);
+ }
+ return data;
+ });
+ }
+ return input;
+}
+function fromLocalWebpack(extensionPath, webpackConfigFileName) {
const result = es.through();
const packagedDependencies = [];
const packageJsonConfig = require(path.join(extensionPath, 'package.json'));
if (packageJsonConfig.dependencies) {
- const webpackRootConfig = require(path.join(extensionPath, 'extension.webpack.config.js'));
+ const webpackRootConfig = require(path.join(extensionPath, webpackConfigFileName));
for (const key in webpackRootConfig.externals) {
if (key in packageJsonConfig.dependencies) {
packagedDependencies.push(key);
@@ -63,30 +97,9 @@ function fromLocalWebpack(extensionPath) {
base: extensionPath,
contents: fs.createReadStream(filePath)
}));
- const filesStream = es.readArray(files);
// check for a webpack configuration files, then invoke webpack
- // and merge its output with the files stream. also rewrite the package.json
- // file to a new entry point
- const webpackConfigLocations = glob.sync(path.join(extensionPath, '/**/extension.webpack.config.js'), { ignore: ['**/node_modules'] });
- const packageJsonFilter = filter(f => {
- if (path.basename(f.path) === 'package.json') {
- // only modify package.json's next to the webpack file.
- // to be safe, use existsSync instead of path comparison.
- return fs.existsSync(path.join(path.dirname(f.path), 'extension.webpack.config.js'));
- }
- return false;
- }, { restore: true });
- const patchFilesStream = filesStream
- .pipe(packageJsonFilter)
- .pipe(buffer())
- .pipe(json((data) => {
- if (data.main) {
- // hardcoded entry point directory!
- data.main = data.main.replace('/out/', /dist/);
- }
- return data;
- }))
- .pipe(packageJsonFilter.restore);
+ // and merge its output with the files stream.
+ const webpackConfigLocations = glob.sync(path.join(extensionPath, '**', webpackConfigFileName), { ignore: ['**/node_modules'] });
const webpackStreams = webpackConfigLocations.map(webpackConfigPath => {
const webpackDone = (err, stats) => {
fancyLog(`Bundled extension: ${ansiColors.yellow(path.join(path.basename(extensionPath), path.relative(extensionPath, webpackConfigPath)))}...`);
@@ -120,7 +133,7 @@ function fromLocalWebpack(extensionPath) {
this.emit('data', data);
}));
});
- es.merge(...webpackStreams, patchFilesStream)
+ es.merge(...webpackStreams, es.readArray(files))
// .pipe(es.through(function (data) {
// // debug
// console.log('out', data.path, data.contents.length);
@@ -184,32 +197,129 @@ const excludedExtensions = [
'vscode-test-resolver',
'ms-vscode.node-debug',
'ms-vscode.node-debug2',
+ 'vscode-notebook-tests',
+ 'vscode-custom-editor-tests',
];
-const builtInExtensions = require('../builtInExtensions.json');
-function packageLocalExtensionsStream() {
- const localExtensionDescriptions = glob.sync('extensions/*/package.json')
+const marketplaceWebExtensions = [
+ 'ms-vscode.references-view'
+];
+const productJson = JSON.parse(fs.readFileSync(path.join(__dirname, '../../product.json'), 'utf8'));
+const builtInExtensions = productJson.builtInExtensions || [];
+const webBuiltInExtensions = productJson.webBuiltInExtensions || [];
+/**
+ * Loosely based on `getExtensionKind` from `src/vs/workbench/services/extensions/common/extensionsUtil.ts`
+ */
+function isWebExtension(manifest) {
+ if (typeof manifest.extensionKind !== 'undefined') {
+ const extensionKind = Array.isArray(manifest.extensionKind) ? manifest.extensionKind : [manifest.extensionKind];
+ return (extensionKind.indexOf('web') >= 0);
+ }
+ return (!Boolean(manifest.main) || Boolean(manifest.browser));
+}
+function packageLocalExtensionsStream(forWeb) {
+ const localExtensionsDescriptions = (glob.sync('extensions/*/package.json')
.map(manifestPath => {
+ const absoluteManifestPath = path.join(root, manifestPath);
const extensionPath = path.dirname(path.join(root, manifestPath));
const extensionName = path.basename(extensionPath);
- return { name: extensionName, path: extensionPath };
+ return { name: extensionName, path: extensionPath, manifestPath: absoluteManifestPath };
})
.filter(({ name }) => excludedExtensions.indexOf(name) === -1)
- .filter(({ name }) => builtInExtensions.every(b => b.name !== name));
- const nodeModules = gulp.src('extensions/node_modules/**', { base: '.' });
- const localExtensions = localExtensionDescriptions.map(extension => {
- return fromLocal(extension.path)
+ .filter(({ name }) => builtInExtensions.every(b => b.name !== name))
+ .filter(({ manifestPath }) => (forWeb ? isWebExtension(require(manifestPath)) : true)));
+ const localExtensionsStream = minifyExtensionResources(es.merge(...localExtensionsDescriptions.map(extension => {
+ return fromLocal(extension.path, forWeb)
.pipe(rename(p => p.dirname = `extensions/${extension.name}/${p.dirname}`));
- });
- return es.merge(nodeModules, ...localExtensions)
- .pipe(util2.setExecutableBit(['**/*.sh']));
+ })));
+ let result;
+ if (forWeb) {
+ result = localExtensionsStream;
+ }
+ else {
+ // also include shared node modules
+ result = es.merge(localExtensionsStream, gulp.src('extensions/node_modules/**', { base: '.' }));
+ }
+ return (result
+ .pipe(util2.setExecutableBit(['**/*.sh'])));
}
exports.packageLocalExtensionsStream = packageLocalExtensionsStream;
-function packageMarketplaceExtensionsStream() {
- const extensions = builtInExtensions.map(extension => {
- return fromMarketplace(extension.name, extension.version, extension.metadata)
+function packageMarketplaceExtensionsStream(forWeb) {
+ const marketplaceExtensionsDescriptions = [
+ ...builtInExtensions.filter(({ name }) => (forWeb ? marketplaceWebExtensions.indexOf(name) >= 0 : true)),
+ ...(forWeb ? webBuiltInExtensions : [])
+ ];
+ const marketplaceExtensionsStream = minifyExtensionResources(es.merge(...marketplaceExtensionsDescriptions
+ .map(extension => {
+ const input = fromMarketplace(extension.name, extension.version, extension.metadata)
.pipe(rename(p => p.dirname = `extensions/${extension.name}/${p.dirname}`));
- });
- return es.merge(extensions)
- .pipe(util2.setExecutableBit(['**/*.sh']));
+ return updateExtensionPackageJSON(input, (data) => {
+ delete data.scripts;
+ delete data.dependencies;
+ delete data.devDependencies;
+ return data;
+ });
+ })));
+ return (marketplaceExtensionsStream
+ .pipe(util2.setExecutableBit(['**/*.sh'])));
}
exports.packageMarketplaceExtensionsStream = packageMarketplaceExtensionsStream;
+function scanBuiltinExtensions(extensionsRoot, exclude = []) {
+ const scannedExtensions = [];
+ try {
+ const extensionsFolders = fs.readdirSync(extensionsRoot);
+ for (const extensionFolder of extensionsFolders) {
+ if (exclude.indexOf(extensionFolder) >= 0) {
+ continue;
+ }
+ const packageJSONPath = path.join(extensionsRoot, extensionFolder, 'package.json');
+ if (!fs.existsSync(packageJSONPath)) {
+ continue;
+ }
+ let packageJSON = JSON.parse(fs.readFileSync(packageJSONPath).toString('utf8'));
+ if (!isWebExtension(packageJSON)) {
+ continue;
+ }
+ const children = fs.readdirSync(path.join(extensionsRoot, extensionFolder));
+ const packageNLSPath = children.filter(child => child === 'package.nls.json')[0];
+ const packageNLS = packageNLSPath ? JSON.parse(fs.readFileSync(path.join(extensionsRoot, extensionFolder, packageNLSPath)).toString()) : undefined;
+ const readme = children.filter(child => /^readme(\.txt|\.md|)$/i.test(child))[0];
+ const changelog = children.filter(child => /^changelog(\.txt|\.md|)$/i.test(child))[0];
+ scannedExtensions.push({
+ extensionPath: extensionFolder,
+ packageJSON,
+ packageNLS,
+ readmePath: readme ? path.join(extensionFolder, readme) : undefined,
+ changelogPath: changelog ? path.join(extensionFolder, changelog) : undefined,
+ });
+ }
+ return scannedExtensions;
+ }
+ catch (ex) {
+ return scannedExtensions;
+ }
+}
+exports.scanBuiltinExtensions = scanBuiltinExtensions;
+function translatePackageJSON(packageJSON, packageNLSPath) {
+ const CharCode_PC = '%'.charCodeAt(0);
+ const packageNls = JSON.parse(fs.readFileSync(packageNLSPath).toString());
+ const translate = (obj) => {
+ for (let key in obj) {
+ const val = obj[key];
+ if (Array.isArray(val)) {
+ val.forEach(translate);
+ }
+ else if (val && typeof val === 'object') {
+ translate(val);
+ }
+ else if (typeof val === 'string' && val.charCodeAt(0) === CharCode_PC && val.charCodeAt(val.length - 1) === CharCode_PC) {
+ const translated = packageNls[val.substr(1, val.length - 2)];
+ if (translated) {
+ obj[key] = translated;
+ }
+ }
+ }
+ };
+ translate(packageJSON);
+ return packageJSON;
+}
+exports.translatePackageJSON = translatePackageJSON;
diff --git a/build/lib/extensions.ts b/build/lib/extensions.ts
index 05bc70948468f..dac71c814798e 100644
--- a/build/lib/extensions.ts
+++ b/build/lib/extensions.ts
@@ -21,6 +21,7 @@ import * as fancyLog from 'fancy-log';
import * as ansiColors from 'ansi-colors';
const buffer = require('gulp-buffer');
import json = require('gulp-json-editor');
+import * as jsoncParser from 'jsonc-parser';
const webpack = require('webpack');
const webpackGulp = require('webpack-stream');
const util = require('./util');
@@ -28,31 +29,67 @@ const root = path.dirname(path.dirname(__dirname));
const commit = util.getVersion(root);
const sourceMappingURLBase = `https://ticino.blob.core.windows.net/sourcemaps/${commit}`;
-function fromLocal(extensionPath: string): Stream {
- const webpackFilename = path.join(extensionPath, 'extension.webpack.config.js');
- const input = fs.existsSync(webpackFilename)
- ? fromLocalWebpack(extensionPath)
- : fromLocalNormal(extensionPath);
-
- const tmLanguageJsonFilter = filter('**/*.tmLanguage.json', { restore: true });
+function minifyExtensionResources(input: Stream): Stream {
+ const jsonFilter = filter(['**/*.json', '**/*.code-snippets'], { restore: true });
+ return input
+ .pipe(jsonFilter)
+ .pipe(buffer())
+ .pipe(es.mapSync((f: File) => {
+ const errors: jsoncParser.ParseError[] = [];
+ const value = jsoncParser.parse(f.contents.toString('utf8'), errors);
+ if (errors.length === 0) {
+ // file parsed OK => just stringify to drop whitespace and comments
+ f.contents = Buffer.from(JSON.stringify(value));
+ }
+ return f;
+ }))
+ .pipe(jsonFilter.restore);
+}
+function updateExtensionPackageJSON(input: Stream, update: (data: any) => any): Stream {
+ const packageJsonFilter = filter('extensions/*/package.json', { restore: true });
return input
- .pipe(tmLanguageJsonFilter)
+ .pipe(packageJsonFilter)
.pipe(buffer())
.pipe(es.mapSync((f: File) => {
- f.contents = Buffer.from(JSON.stringify(JSON.parse(f.contents.toString('utf8'))));
+ const data = JSON.parse(f.contents.toString('utf8'));
+ f.contents = Buffer.from(JSON.stringify(update(data)));
return f;
}))
- .pipe(tmLanguageJsonFilter.restore);
+ .pipe(packageJsonFilter.restore);
+}
+
+function fromLocal(extensionPath: string, forWeb: boolean): Stream {
+ const webpackConfigFileName = forWeb ? 'extension-browser.webpack.config.js' : 'extension.webpack.config.js';
+
+ const isWebPacked = fs.existsSync(path.join(extensionPath, webpackConfigFileName));
+ let input = isWebPacked
+ ? fromLocalWebpack(extensionPath, webpackConfigFileName)
+ : fromLocalNormal(extensionPath);
+
+ if (isWebPacked) {
+ input = updateExtensionPackageJSON(input, (data: any) => {
+ delete data.scripts;
+ delete data.dependencies;
+ delete data.devDependencies;
+ if (data.main) {
+ data.main = data.main.replace('/out/', /dist/);
+ }
+ return data;
+ });
+ }
+
+ return input;
}
-function fromLocalWebpack(extensionPath: string): Stream {
+
+function fromLocalWebpack(extensionPath: string, webpackConfigFileName: string): Stream {
const result = es.through();
const packagedDependencies: string[] = [];
const packageJsonConfig = require(path.join(extensionPath, 'package.json'));
if (packageJsonConfig.dependencies) {
- const webpackRootConfig = require(path.join(extensionPath, 'extension.webpack.config.js'));
+ const webpackRootConfig = require(path.join(extensionPath, webpackConfigFileName));
for (const key in webpackRootConfig.externals) {
if (key in packageJsonConfig.dependencies) {
packagedDependencies.push(key);
@@ -70,38 +107,13 @@ function fromLocalWebpack(extensionPath: string): Stream {
contents: fs.createReadStream(filePath) as any
}));
- const filesStream = es.readArray(files);
-
// check for a webpack configuration files, then invoke webpack
- // and merge its output with the files stream. also rewrite the package.json
- // file to a new entry point
+ // and merge its output with the files stream.
const webpackConfigLocations = (glob.sync(
- path.join(extensionPath, '/**/extension.webpack.config.js'),
+ path.join(extensionPath, '**', webpackConfigFileName),
{ ignore: ['**/node_modules'] }
));
- const packageJsonFilter = filter(f => {
- if (path.basename(f.path) === 'package.json') {
- // only modify package.json's next to the webpack file.
- // to be safe, use existsSync instead of path comparison.
- return fs.existsSync(path.join(path.dirname(f.path), 'extension.webpack.config.js'));
- }
- return false;
- }, { restore: true });
-
- const patchFilesStream = filesStream
- .pipe(packageJsonFilter)
- .pipe(buffer())
- .pipe(json((data: any) => {
- if (data.main) {
- // hardcoded entry point directory!
- data.main = data.main.replace('/out/', /dist/);
- }
- return data;
- }))
- .pipe(packageJsonFilter.restore);
-
-
const webpackStreams = webpackConfigLocations.map(webpackConfigPath => {
const webpackDone = (err: any, stats: any) => {
@@ -143,7 +155,7 @@ function fromLocalWebpack(extensionPath: string): Stream {
}));
});
- es.merge(...webpackStreams, patchFilesStream)
+ es.merge(...webpackStreams, es.readArray(files))
// .pipe(es.through(function (data) {
// // debug
// console.log('out', data.path, data.contents.length);
@@ -212,13 +224,18 @@ export function fromMarketplace(extensionName: string, version: string, metadata
.pipe(json({ __metadata: metadata }))
.pipe(packageJsonFilter.restore);
}
-
const excludedExtensions = [
'vscode-api-tests',
'vscode-colorize-tests',
'vscode-test-resolver',
'ms-vscode.node-debug',
'ms-vscode.node-debug2',
+ 'vscode-notebook-tests',
+ 'vscode-custom-editor-tests',
+];
+
+const marketplaceWebExtensions = [
+ 'ms-vscode.references-view'
];
interface IBuiltInExtension {
@@ -228,34 +245,153 @@ interface IBuiltInExtension {
metadata: any;
}
-const builtInExtensions: IBuiltInExtension[] = require('../builtInExtensions.json');
+const productJson = JSON.parse(fs.readFileSync(path.join(__dirname, '../../product.json'), 'utf8'));
+const builtInExtensions: IBuiltInExtension[] = productJson.builtInExtensions || [];
+const webBuiltInExtensions: IBuiltInExtension[] = productJson.webBuiltInExtensions || [];
-export function packageLocalExtensionsStream(): NodeJS.ReadWriteStream {
- const localExtensionDescriptions = (glob.sync('extensions/*/package.json'))
- .map(manifestPath => {
- const extensionPath = path.dirname(path.join(root, manifestPath));
- const extensionName = path.basename(extensionPath);
- return { name: extensionName, path: extensionPath };
- })
- .filter(({ name }) => excludedExtensions.indexOf(name) === -1)
- .filter(({ name }) => builtInExtensions.every(b => b.name !== name));
+type ExtensionKind = 'ui' | 'workspace' | 'web';
+interface IExtensionManifest {
+ main: string;
+ browser: string;
+ extensionKind?: ExtensionKind | ExtensionKind[];
+}
+/**
+ * Loosely based on `getExtensionKind` from `src/vs/workbench/services/extensions/common/extensionsUtil.ts`
+ */
+function isWebExtension(manifest: IExtensionManifest): boolean {
+ if (typeof manifest.extensionKind !== 'undefined') {
+ const extensionKind = Array.isArray(manifest.extensionKind) ? manifest.extensionKind : [manifest.extensionKind];
+ return (extensionKind.indexOf('web') >= 0);
+ }
+ return (!Boolean(manifest.main) || Boolean(manifest.browser));
+}
- const nodeModules = gulp.src('extensions/node_modules/**', { base: '.' });
- const localExtensions = localExtensionDescriptions.map(extension => {
- return fromLocal(extension.path)
- .pipe(rename(p => p.dirname = `extensions/${extension.name}/${p.dirname}`));
- });
+export function packageLocalExtensionsStream(forWeb: boolean): Stream {
+ const localExtensionsDescriptions = (
+ (glob.sync('extensions/*/package.json'))
+ .map(manifestPath => {
+ const absoluteManifestPath = path.join(root, manifestPath);
+ const extensionPath = path.dirname(path.join(root, manifestPath));
+ const extensionName = path.basename(extensionPath);
+ return { name: extensionName, path: extensionPath, manifestPath: absoluteManifestPath };
+ })
+ .filter(({ name }) => excludedExtensions.indexOf(name) === -1)
+ .filter(({ name }) => builtInExtensions.every(b => b.name !== name))
+ .filter(({ manifestPath }) => (forWeb ? isWebExtension(require(manifestPath)) : true))
+ );
+ const localExtensionsStream = minifyExtensionResources(
+ es.merge(
+ ...localExtensionsDescriptions.map(extension => {
+ return fromLocal(extension.path, forWeb)
+ .pipe(rename(p => p.dirname = `extensions/${extension.name}/${p.dirname}`));
+ })
+ )
+ );
+
+ let result: Stream;
+ if (forWeb) {
+ result = localExtensionsStream;
+ } else {
+ // also include shared node modules
+ result = es.merge(localExtensionsStream, gulp.src('extensions/node_modules/**', { base: '.' }));
+ }
- return es.merge(nodeModules, ...localExtensions)
- .pipe(util2.setExecutableBit(['**/*.sh']));
+ return (
+ result
+ .pipe(util2.setExecutableBit(['**/*.sh']))
+ );
}
-export function packageMarketplaceExtensionsStream(): NodeJS.ReadWriteStream {
- const extensions = builtInExtensions.map(extension => {
- return fromMarketplace(extension.name, extension.version, extension.metadata)
- .pipe(rename(p => p.dirname = `extensions/${extension.name}/${p.dirname}`));
- });
+export function packageMarketplaceExtensionsStream(forWeb: boolean): Stream {
+ const marketplaceExtensionsDescriptions = [
+ ...builtInExtensions.filter(({ name }) => (forWeb ? marketplaceWebExtensions.indexOf(name) >= 0 : true)),
+ ...(forWeb ? webBuiltInExtensions : [])
+ ];
+ const marketplaceExtensionsStream = minifyExtensionResources(
+ es.merge(
+ ...marketplaceExtensionsDescriptions
+ .map(extension => {
+ const input = fromMarketplace(extension.name, extension.version, extension.metadata)
+ .pipe(rename(p => p.dirname = `extensions/${extension.name}/${p.dirname}`));
+ return updateExtensionPackageJSON(input, (data: any) => {
+ delete data.scripts;
+ delete data.dependencies;
+ delete data.devDependencies;
+ return data;
+ });
+ })
+ )
+ );
+
+ return (
+ marketplaceExtensionsStream
+ .pipe(util2.setExecutableBit(['**/*.sh']))
+ );
+}
+
+export interface IScannedBuiltinExtension {
+ extensionPath: string;
+ packageJSON: any;
+ packageNLS?: any;
+ readmePath?: string;
+ changelogPath?: string;
+}
- return es.merge(extensions)
- .pipe(util2.setExecutableBit(['**/*.sh']));
+export function scanBuiltinExtensions(extensionsRoot: string, exclude: string[] = []): IScannedBuiltinExtension[] {
+ const scannedExtensions: IScannedBuiltinExtension[] = [];
+
+ try {
+ const extensionsFolders = fs.readdirSync(extensionsRoot);
+ for (const extensionFolder of extensionsFolders) {
+ if (exclude.indexOf(extensionFolder) >= 0) {
+ continue;
+ }
+ const packageJSONPath = path.join(extensionsRoot, extensionFolder, 'package.json');
+ if (!fs.existsSync(packageJSONPath)) {
+ continue;
+ }
+ let packageJSON = JSON.parse(fs.readFileSync(packageJSONPath).toString('utf8'));
+ if (!isWebExtension(packageJSON)) {
+ continue;
+ }
+ const children = fs.readdirSync(path.join(extensionsRoot, extensionFolder));
+ const packageNLSPath = children.filter(child => child === 'package.nls.json')[0];
+ const packageNLS = packageNLSPath ? JSON.parse(fs.readFileSync(path.join(extensionsRoot, extensionFolder, packageNLSPath)).toString()) : undefined;
+ const readme = children.filter(child => /^readme(\.txt|\.md|)$/i.test(child))[0];
+ const changelog = children.filter(child => /^changelog(\.txt|\.md|)$/i.test(child))[0];
+
+ scannedExtensions.push({
+ extensionPath: extensionFolder,
+ packageJSON,
+ packageNLS,
+ readmePath: readme ? path.join(extensionFolder, readme) : undefined,
+ changelogPath: changelog ? path.join(extensionFolder, changelog) : undefined,
+ });
+ }
+ return scannedExtensions;
+ } catch (ex) {
+ return scannedExtensions;
+ }
+}
+
+export function translatePackageJSON(packageJSON: string, packageNLSPath: string) {
+ const CharCode_PC = '%'.charCodeAt(0);
+ const packageNls = JSON.parse(fs.readFileSync(packageNLSPath).toString());
+ const translate = (obj: any) => {
+ for (let key in obj) {
+ const val = obj[key];
+ if (Array.isArray(val)) {
+ val.forEach(translate);
+ } else if (val && typeof val === 'object') {
+ translate(val);
+ } else if (typeof val === 'string' && val.charCodeAt(0) === CharCode_PC && val.charCodeAt(val.length - 1) === CharCode_PC) {
+ const translated = packageNls[val.substr(1, val.length - 2)];
+ if (translated) {
+ obj[key] = translated;
+ }
+ }
+ }
+ };
+ translate(packageJSON);
+ return packageJSON;
}
diff --git a/build/lib/git.js b/build/lib/git.js
index da5d66fd8d222..1726f76fcc791 100644
--- a/build/lib/git.js
+++ b/build/lib/git.js
@@ -4,6 +4,7 @@
*--------------------------------------------------------------------------------------------*/
'use strict';
Object.defineProperty(exports, "__esModule", { value: true });
+exports.getVersion = void 0;
const path = require("path");
const fs = require("fs");
/**
diff --git a/build/lib/i18n.js b/build/lib/i18n.js
index 27a4054a1e4d0..7371b0f022f8a 100644
--- a/build/lib/i18n.js
+++ b/build/lib/i18n.js
@@ -4,6 +4,7 @@
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
Object.defineProperty(exports, "__esModule", { value: true });
+exports.prepareIslFiles = exports.prepareI18nPackFiles = exports.pullI18nPackFiles = exports.prepareI18nFiles = exports.pullSetupXlfFiles = exports.pullCoreAndExtensionsXlfFiles = exports.findObsoleteResources = exports.pushXlfFiles = exports.createXlfFilesForIsl = exports.createXlfFilesForExtensions = exports.createXlfFilesForCoreBundle = exports.getResource = exports.processNlsFiles = exports.Limiter = exports.XLF = exports.Line = exports.externalExtensionsWithTranslations = exports.extraLanguages = exports.defaultLanguages = void 0;
const path = require("path");
const fs = require("fs");
const event_stream_1 = require("event-stream");
@@ -15,7 +16,7 @@ const https = require("https");
const gulp = require("gulp");
const fancyLog = require("fancy-log");
const ansiColors = require("ansi-colors");
-const iconv = require("iconv-lite");
+const iconv = require("iconv-lite-umd");
const NUMBER_OF_CONCURRENT_DOWNLOADS = 4;
function log(message, ...rest) {
fancyLog(ansiColors.green('[i18n]'), message, ...rest);
@@ -112,7 +113,7 @@ class XLF {
for (let file in this.files) {
this.appendNewLine(``, 2);
for (let item of this.files[file]) {
- this.addStringItem(item);
+ this.addStringItem(file, item);
}
this.appendNewLine('', 2);
}
@@ -152,9 +153,12 @@ class XLF {
this.files[original].push({ id: realKey, message: message, comment: comment });
}
}
- addStringItem(item) {
- if (!item.id || !item.message) {
- throw new Error(`No item ID or value specified: ${JSON.stringify(item)}`);
+ addStringItem(file, item) {
+ if (!item.id || item.message === undefined || item.message === null) {
+ throw new Error(`No item ID or value specified: ${JSON.stringify(item)}. File: ${file}`);
+ }
+ if (item.message.length === 0) {
+ log(`Item with id ${item.id} in file ${file} has an empty message.`);
}
this.appendNewLine(``, 4);
this.appendNewLine(`${item.message}`, 6);
@@ -1137,12 +1141,7 @@ function createIslFile(originalFilePath, messages, language, innoSetup) {
if (line.length > 0) {
let firstChar = line.charAt(0);
if (firstChar === '[' || firstChar === ';') {
- if (line === '; *** Inno Setup version 5.5.3+ English messages ***') {
- content.push(`; *** Inno Setup version 5.5.3+ ${innoSetup.defaultInfo.name} messages ***`);
- }
- else {
- content.push(line);
- }
+ content.push(line);
}
else {
let sections = line.split('=');
@@ -1171,9 +1170,10 @@ function createIslFile(originalFilePath, messages, language, innoSetup) {
});
const basename = path.basename(originalFilePath);
const filePath = `${basename}.${language.id}.isl`;
+ const encoded = iconv.encode(Buffer.from(content.join('\r\n'), 'utf8').toString(), innoSetup.codePage);
return new File({
path: filePath,
- contents: iconv.encode(Buffer.from(content.join('\r\n'), 'utf8').toString(), innoSetup.codePage)
+ contents: Buffer.from(encoded),
});
}
function encodeEntities(value) {
diff --git a/build/lib/i18n.resources.json b/build/lib/i18n.resources.json
index 2e2a20d89adbf..a192a31e57dae 100644
--- a/build/lib/i18n.resources.json
+++ b/build/lib/i18n.resources.json
@@ -30,6 +30,14 @@
"name": "vs/workbench/api/common",
"project": "vscode-workbench"
},
+ {
+ "name": "vs/workbench/contrib/backup",
+ "project": "vscode-workbench"
+ },
+ {
+ "name": "vs/workbench/contrib/bulkEdit",
+ "project": "vscode-workbench"
+ },
{
"name": "vs/workbench/contrib/cli",
"project": "vscode-workbench"
@@ -58,6 +66,10 @@
"name": "vs/workbench/contrib/emmet",
"project": "vscode-workbench"
},
+ {
+ "name": "vs/workbench/contrib/experiments",
+ "project": "vscode-workbench"
+ },
{
"name": "vs/workbench/contrib/extensions",
"project": "vscode-workbench"
@@ -82,6 +94,10 @@
"name": "vs/workbench/contrib/issue",
"project": "vscode-workbench"
},
+ {
+ "name": "vs/workbench/contrib/keybindings",
+ "project": "vscode-workbench"
+ },
{
"name": "vs/workbench/contrib/markers",
"project": "vscode-workbench"
@@ -107,7 +123,11 @@
"project": "vscode-workbench"
},
{
- "name": "vs/workbench/contrib/quickopen",
+ "name": "vs/workbench/contrib/notebook",
+ "project": "vscode-workbench"
+ },
+ {
+ "name": "vs/workbench/contrib/quickaccess",
"project": "vscode-workbench"
},
{
@@ -122,6 +142,10 @@
"name": "vs/workbench/contrib/relauncher",
"project": "vscode-workbench"
},
+ {
+ "name": "vs/workbench/contrib/sash",
+ "project": "vscode-workbench"
+ },
{
"name": "vs/workbench/contrib/scm",
"project": "vscode-workbench"
@@ -130,6 +154,10 @@
"name": "vs/workbench/contrib/search",
"project": "vscode-workbench"
},
+ {
+ "name": "vs/workbench/contrib/searchEditor",
+ "project": "vscode-workbench"
+ },
{
"name": "vs/workbench/contrib/snippets",
"project": "vscode-workbench"
@@ -139,7 +167,7 @@
"project": "vscode-workbench"
},
{
- "name": "vs/workbench/contrib/stats",
+ "name": "vs/workbench/contrib/tags",
"project": "vscode-workbench"
},
{
@@ -194,10 +222,18 @@
"name": "vs/workbench/contrib/userDataSync",
"project": "vscode-workbench"
},
+ {
+ "name": "vs/workbench/contrib/views",
+ "project": "vscode-workbench"
+ },
{
"name": "vs/workbench/services/actions",
"project": "vscode-workbench"
},
+ {
+ "name": "vs/workbench/services/authToken",
+ "project": "vscode-workbench"
+ },
{
"name": "vs/workbench/services/bulkEdit",
"project": "vscode-workbench"
@@ -214,10 +250,6 @@
"name": "vs/workbench/services/configurationResolver",
"project": "vscode-workbench"
},
- {
- "name": "vs/workbench/services/crashReporter",
- "project": "vscode-workbench"
- },
{
"name": "vs/workbench/services/dialogs",
"project": "vscode-workbench"
@@ -238,6 +270,10 @@
"name": "vs/workbench/services/files",
"project": "vscode-workbench"
},
+ {
+ "name": "vs/workbench/services/log",
+ "project": "vscode-workbench"
+ },
{
"name": "vs/workbench/services/integrity",
"project": "vscode-workbench"
@@ -262,6 +298,10 @@
"name": "vs/workbench/services/remote",
"project": "vscode-workbench"
},
+ {
+ "name": "vs/workbench/services/search",
+ "project": "vscode-workbench"
+ },
{
"name": "vs/workbench/services/textfile",
"project": "vscode-workbench"
@@ -274,6 +314,10 @@
"name": "vs/workbench/services/textMate",
"project": "vscode-workbench"
},
+ {
+ "name": "vs/workbench/services/workingCopy",
+ "project": "vscode-workbench"
+ },
{
"name": "vs/workbench/services/workspaces",
"project": "vscode-workbench"
@@ -297,6 +341,22 @@
{
"name": "vs/workbench/services/userData",
"project": "vscode-workbench"
+ },
+ {
+ "name": "vs/workbench/services/userDataSync",
+ "project": "vscode-workbench"
+ },
+ {
+ "name": "vs/workbench/services/views",
+ "project": "vscode-workbench"
+ },
+ {
+ "name": "vs/workbench/contrib/timeline",
+ "project": "vscode-workbench"
+ },
+ {
+ "name": "vs/workbench/services/authentication",
+ "project": "vscode-workbench"
}
]
}
diff --git a/build/lib/i18n.ts b/build/lib/i18n.ts
index d69109a9d05b4..418a7b5c362ab 100644
--- a/build/lib/i18n.ts
+++ b/build/lib/i18n.ts
@@ -15,7 +15,7 @@ import * as https from 'https';
import * as gulp from 'gulp';
import * as fancyLog from 'fancy-log';
import * as ansiColors from 'ansi-colors';
-import * as iconv from 'iconv-lite';
+import * as iconv from 'iconv-lite-umd';
const NUMBER_OF_CONCURRENT_DOWNLOADS = 4;
@@ -201,7 +201,7 @@ export class XLF {
for (let file in this.files) {
this.appendNewLine(``, 2);
for (let item of this.files[file]) {
- this.addStringItem(item);
+ this.addStringItem(file, item);
}
this.appendNewLine('', 2);
}
@@ -243,9 +243,12 @@ export class XLF {
}
}
- private addStringItem(item: Item): void {
- if (!item.id || !item.message) {
- throw new Error(`No item ID or value specified: ${JSON.stringify(item)}`);
+ private addStringItem(file: string, item: Item): void {
+ if (!item.id || item.message === undefined || item.message === null) {
+ throw new Error(`No item ID or value specified: ${JSON.stringify(item)}. File: ${file}`);
+ }
+ if (item.message.length === 0) {
+ log(`Item with id ${item.id} in file ${file} has an empty message.`);
}
this.appendNewLine(``, 4);
@@ -993,7 +996,7 @@ function createResource(project: string, slug: string, xlfFile: File, apiHostnam
* https://dev.befoolish.co/tx-docs/public/projects/updating-content#what-happens-when-you-update-files
*/
function updateResource(project: string, slug: string, xlfFile: File, apiHostname: string, credentials: string): Promise {
- return new Promise((resolve, reject) => {
+ return new Promise((resolve, reject) => {
const data = JSON.stringify({ content: xlfFile.contents.toString() });
const options = {
hostname: apiHostname,
@@ -1305,11 +1308,7 @@ function createIslFile(originalFilePath: string, messages: Map, language
if (line.length > 0) {
let firstChar = line.charAt(0);
if (firstChar === '[' || firstChar === ';') {
- if (line === '; *** Inno Setup version 5.5.3+ English messages ***') {
- content.push(`; *** Inno Setup version 5.5.3+ ${innoSetup.defaultInfo!.name} messages ***`);
- } else {
- content.push(line);
- }
+ content.push(line);
} else {
let sections: string[] = line.split('=');
let key = sections[0];
@@ -1336,10 +1335,11 @@ function createIslFile(originalFilePath: string, messages: Map, language
const basename = path.basename(originalFilePath);
const filePath = `${basename}.${language.id}.isl`;
+ const encoded = iconv.encode(Buffer.from(content.join('\r\n'), 'utf8').toString(), innoSetup.codePage);
return new File({
path: filePath,
- contents: iconv.encode(Buffer.from(content.join('\r\n'), 'utf8').toString(), innoSetup.codePage)
+ contents: Buffer.from(encoded),
});
}
diff --git a/build/lib/layersChecker.js b/build/lib/layersChecker.js
new file mode 100644
index 0000000000000..864c47f6ca3a0
--- /dev/null
+++ b/build/lib/layersChecker.js
@@ -0,0 +1,274 @@
+"use strict";
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+Object.defineProperty(exports, "__esModule", { value: true });
+const ts = require("typescript");
+const fs_1 = require("fs");
+const path_1 = require("path");
+const minimatch_1 = require("minimatch");
+//
+// #############################################################################################
+//
+// A custom typescript checker for the specific task of detecting the use of certain types in a
+// layer that does not allow such use. For example:
+// - using DOM globals in common/node/electron-main layer (e.g. HTMLElement)
+// - using node.js globals in common/browser layer (e.g. process)
+//
+// Make changes to below RULES to lift certain files from these checks only if absolutely needed
+//
+// #############################################################################################
+//
+// Types we assume are present in all implementations of JS VMs (node.js, browsers)
+// Feel free to add more core types as you see needed if present in node.js and browsers
+const CORE_TYPES = [
+ 'require',
+ 'atob',
+ 'btoa',
+ 'setTimeout',
+ 'clearTimeout',
+ 'setInterval',
+ 'clearInterval',
+ 'console',
+ 'log',
+ 'info',
+ 'warn',
+ 'error',
+ 'group',
+ 'groupEnd',
+ 'table',
+ 'assert',
+ 'Error',
+ 'String',
+ 'throws',
+ 'stack',
+ 'captureStackTrace',
+ 'stackTraceLimit',
+ 'TextDecoder',
+ 'TextEncoder',
+ 'encode',
+ 'decode',
+ 'self',
+ 'trimLeft',
+ 'trimRight'
+];
+// Types that are defined in a common layer but are known to be only
+// available in native environments should not be allowed in browser
+const NATIVE_TYPES = [
+ 'NativeParsedArgs',
+ 'INativeEnvironmentService',
+ 'INativeWindowConfiguration'
+];
+const RULES = [
+ // Tests: skip
+ {
+ target: '**/vs/**/test/**',
+ skip: true // -> skip all test files
+ },
+ // Common: vs/base/common/platform.ts
+ {
+ target: '**/vs/base/common/platform.ts',
+ allowedTypes: [
+ ...CORE_TYPES,
+ // Safe access to postMessage() and friends
+ 'MessageEvent',
+ 'data'
+ ],
+ disallowedTypes: NATIVE_TYPES,
+ disallowedDefinitions: [
+ 'lib.dom.d.ts',
+ '@types/node' // no node.js
+ ]
+ },
+ // Common: vs/platform/environment/common/argv.ts
+ {
+ target: '**/vs/platform/environment/common/argv.ts',
+ disallowedTypes: [ /* Ignore native types that are defined from here */],
+ allowedTypes: CORE_TYPES,
+ disallowedDefinitions: [
+ 'lib.dom.d.ts',
+ '@types/node' // no node.js
+ ]
+ },
+ // Common: vs/platform/environment/common/environment.ts
+ {
+ target: '**/vs/platform/environment/common/environment.ts',
+ disallowedTypes: [ /* Ignore native types that are defined from here */],
+ allowedTypes: CORE_TYPES,
+ disallowedDefinitions: [
+ 'lib.dom.d.ts',
+ '@types/node' // no node.js
+ ]
+ },
+ // Common: vs/platform/windows/common/windows.ts
+ {
+ target: '**/vs/platform/windows/common/windows.ts',
+ disallowedTypes: [ /* Ignore native types that are defined from here */],
+ allowedTypes: CORE_TYPES,
+ disallowedDefinitions: [
+ 'lib.dom.d.ts',
+ '@types/node' // no node.js
+ ]
+ },
+ // Common: vs/workbench/api/common/extHostExtensionService.ts
+ {
+ target: '**/vs/workbench/api/common/extHostExtensionService.ts',
+ allowedTypes: [
+ ...CORE_TYPES,
+ // Safe access to global
+ 'global'
+ ],
+ disallowedTypes: NATIVE_TYPES,
+ disallowedDefinitions: [
+ 'lib.dom.d.ts',
+ '@types/node' // no node.js
+ ]
+ },
+ // Common
+ {
+ target: '**/vs/**/common/**',
+ allowedTypes: CORE_TYPES,
+ disallowedTypes: NATIVE_TYPES,
+ disallowedDefinitions: [
+ 'lib.dom.d.ts',
+ '@types/node' // no node.js
+ ]
+ },
+ // Browser
+ {
+ target: '**/vs/**/browser/**',
+ allowedTypes: CORE_TYPES,
+ disallowedTypes: NATIVE_TYPES,
+ disallowedDefinitions: [
+ '@types/node' // no node.js
+ ]
+ },
+ // Browser (editor contrib)
+ {
+ target: '**/src/vs/editor/contrib/**',
+ allowedTypes: CORE_TYPES,
+ disallowedTypes: NATIVE_TYPES,
+ disallowedDefinitions: [
+ '@types/node' // no node.js
+ ]
+ },
+ // node.js
+ {
+ target: '**/vs/**/node/**',
+ allowedTypes: [
+ ...CORE_TYPES,
+ // --> types from node.d.ts that duplicate from lib.dom.d.ts
+ 'URL',
+ 'protocol',
+ 'hostname',
+ 'port',
+ 'pathname',
+ 'search',
+ 'username',
+ 'password'
+ ],
+ disallowedDefinitions: [
+ 'lib.dom.d.ts' // no DOM
+ ]
+ },
+ // Electron (sandbox)
+ {
+ target: '**/vs/**/electron-sandbox/**',
+ allowedTypes: CORE_TYPES,
+ disallowedDefinitions: [
+ '@types/node' // no node.js
+ ]
+ },
+ // Electron (renderer): skip
+ {
+ target: '**/vs/**/electron-browser/**',
+ skip: true // -> supports all types
+ },
+ // Electron (main)
+ {
+ target: '**/vs/**/electron-main/**',
+ allowedTypes: [
+ ...CORE_TYPES,
+ // --> types from electron.d.ts that duplicate from lib.dom.d.ts
+ 'Event',
+ 'Request'
+ ],
+ disallowedDefinitions: [
+ 'lib.dom.d.ts' // no DOM
+ ]
+ }
+];
+const TS_CONFIG_PATH = path_1.join(__dirname, '../../', 'src', 'tsconfig.json');
+let hasErrors = false;
+function checkFile(program, sourceFile, rule) {
+ checkNode(sourceFile);
+ function checkNode(node) {
+ var _a, _b;
+ if (node.kind !== ts.SyntaxKind.Identifier) {
+ return ts.forEachChild(node, checkNode); // recurse down
+ }
+ const text = node.getText(sourceFile);
+ if ((_a = rule.allowedTypes) === null || _a === void 0 ? void 0 : _a.some(allowed => allowed === text)) {
+ return; // override
+ }
+ if ((_b = rule.disallowedTypes) === null || _b === void 0 ? void 0 : _b.some(disallowed => disallowed === text)) {
+ const { line, character } = sourceFile.getLineAndCharacterOfPosition(node.getStart());
+ console.log(`[build/lib/layersChecker.ts]: Reference to '${text}' violates layer '${rule.target}' (${sourceFile.fileName} (${line + 1},${character + 1})`);
+ hasErrors = true;
+ return;
+ }
+ const checker = program.getTypeChecker();
+ const symbol = checker.getSymbolAtLocation(node);
+ if (symbol) {
+ const declarations = symbol.declarations;
+ if (Array.isArray(declarations)) {
+ for (const declaration of declarations) {
+ if (declaration) {
+ const parent = declaration.parent;
+ if (parent) {
+ const parentSourceFile = parent.getSourceFile();
+ if (parentSourceFile) {
+ const definitionFileName = parentSourceFile.fileName;
+ if (rule.disallowedDefinitions) {
+ for (const disallowedDefinition of rule.disallowedDefinitions) {
+ if (definitionFileName.indexOf(disallowedDefinition) >= 0) {
+ const { line, character } = sourceFile.getLineAndCharacterOfPosition(node.getStart());
+ console.log(`[build/lib/layersChecker.ts]: Reference to '${text}' from '${disallowedDefinition}' violates layer '${rule.target}' (${sourceFile.fileName} (${line + 1},${character + 1})`);
+ hasErrors = true;
+ return;
+ }
+ }
+ }
+ }
+ }
+ }
+ }
+ }
+ }
+ }
+}
+function createProgram(tsconfigPath) {
+ const tsConfig = ts.readConfigFile(tsconfigPath, ts.sys.readFile);
+ const configHostParser = { fileExists: fs_1.existsSync, readDirectory: ts.sys.readDirectory, readFile: file => fs_1.readFileSync(file, 'utf8'), useCaseSensitiveFileNames: process.platform === 'linux' };
+ const tsConfigParsed = ts.parseJsonConfigFileContent(tsConfig.config, configHostParser, path_1.resolve(path_1.dirname(tsconfigPath)), { noEmit: true });
+ const compilerHost = ts.createCompilerHost(tsConfigParsed.options, true);
+ return ts.createProgram(tsConfigParsed.fileNames, tsConfigParsed.options, compilerHost);
+}
+//
+// Create program and start checking
+//
+const program = createProgram(TS_CONFIG_PATH);
+for (const sourceFile of program.getSourceFiles()) {
+ for (const rule of RULES) {
+ if (minimatch_1.match([sourceFile.fileName], rule.target).length > 0) {
+ if (!rule.skip) {
+ checkFile(program, sourceFile, rule);
+ }
+ break;
+ }
+ }
+}
+if (hasErrors) {
+ process.exit(1);
+}
diff --git a/build/lib/layersChecker.ts b/build/lib/layersChecker.ts
new file mode 100644
index 0000000000000..5d620c79db038
--- /dev/null
+++ b/build/lib/layersChecker.ts
@@ -0,0 +1,319 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import * as ts from 'typescript';
+import { readFileSync, existsSync } from 'fs';
+import { resolve, dirname, join } from 'path';
+import { match } from 'minimatch';
+
+//
+// #############################################################################################
+//
+// A custom typescript checker for the specific task of detecting the use of certain types in a
+// layer that does not allow such use. For example:
+// - using DOM globals in common/node/electron-main layer (e.g. HTMLElement)
+// - using node.js globals in common/browser layer (e.g. process)
+//
+// Make changes to below RULES to lift certain files from these checks only if absolutely needed
+//
+// #############################################################################################
+//
+
+// Types we assume are present in all implementations of JS VMs (node.js, browsers)
+// Feel free to add more core types as you see needed if present in node.js and browsers
+const CORE_TYPES = [
+ 'require', // from our AMD loader
+ 'atob',
+ 'btoa',
+ 'setTimeout',
+ 'clearTimeout',
+ 'setInterval',
+ 'clearInterval',
+ 'console',
+ 'log',
+ 'info',
+ 'warn',
+ 'error',
+ 'group',
+ 'groupEnd',
+ 'table',
+ 'assert',
+ 'Error',
+ 'String',
+ 'throws',
+ 'stack',
+ 'captureStackTrace',
+ 'stackTraceLimit',
+ 'TextDecoder',
+ 'TextEncoder',
+ 'encode',
+ 'decode',
+ 'self',
+ 'trimLeft',
+ 'trimRight'
+];
+
+// Types that are defined in a common layer but are known to be only
+// available in native environments should not be allowed in browser
+const NATIVE_TYPES = [
+ 'NativeParsedArgs',
+ 'INativeEnvironmentService',
+ 'INativeWindowConfiguration'
+];
+
+const RULES = [
+
+ // Tests: skip
+ {
+ target: '**/vs/**/test/**',
+ skip: true // -> skip all test files
+ },
+
+ // Common: vs/base/common/platform.ts
+ {
+ target: '**/vs/base/common/platform.ts',
+ allowedTypes: [
+ ...CORE_TYPES,
+
+ // Safe access to postMessage() and friends
+ 'MessageEvent',
+ 'data'
+ ],
+ disallowedTypes: NATIVE_TYPES,
+ disallowedDefinitions: [
+ 'lib.dom.d.ts', // no DOM
+ '@types/node' // no node.js
+ ]
+ },
+
+ // Common: vs/platform/environment/common/argv.ts
+ {
+ target: '**/vs/platform/environment/common/argv.ts',
+ disallowedTypes: [/* Ignore native types that are defined from here */],
+ allowedTypes: CORE_TYPES,
+ disallowedDefinitions: [
+ 'lib.dom.d.ts', // no DOM
+ '@types/node' // no node.js
+ ]
+ },
+
+ // Common: vs/platform/environment/common/environment.ts
+ {
+ target: '**/vs/platform/environment/common/environment.ts',
+ disallowedTypes: [/* Ignore native types that are defined from here */],
+ allowedTypes: CORE_TYPES,
+ disallowedDefinitions: [
+ 'lib.dom.d.ts', // no DOM
+ '@types/node' // no node.js
+ ]
+ },
+
+ // Common: vs/platform/windows/common/windows.ts
+ {
+ target: '**/vs/platform/windows/common/windows.ts',
+ disallowedTypes: [/* Ignore native types that are defined from here */],
+ allowedTypes: CORE_TYPES,
+ disallowedDefinitions: [
+ 'lib.dom.d.ts', // no DOM
+ '@types/node' // no node.js
+ ]
+ },
+
+ // Common: vs/workbench/api/common/extHostExtensionService.ts
+ {
+ target: '**/vs/workbench/api/common/extHostExtensionService.ts',
+ allowedTypes: [
+ ...CORE_TYPES,
+
+ // Safe access to global
+ 'global'
+ ],
+ disallowedTypes: NATIVE_TYPES,
+ disallowedDefinitions: [
+ 'lib.dom.d.ts', // no DOM
+ '@types/node' // no node.js
+ ]
+ },
+
+ // Common
+ {
+ target: '**/vs/**/common/**',
+ allowedTypes: CORE_TYPES,
+ disallowedTypes: NATIVE_TYPES,
+ disallowedDefinitions: [
+ 'lib.dom.d.ts', // no DOM
+ '@types/node' // no node.js
+ ]
+ },
+
+ // Browser
+ {
+ target: '**/vs/**/browser/**',
+ allowedTypes: CORE_TYPES,
+ disallowedTypes: NATIVE_TYPES,
+ disallowedDefinitions: [
+ '@types/node' // no node.js
+ ]
+ },
+
+ // Browser (editor contrib)
+ {
+ target: '**/src/vs/editor/contrib/**',
+ allowedTypes: CORE_TYPES,
+ disallowedTypes: NATIVE_TYPES,
+ disallowedDefinitions: [
+ '@types/node' // no node.js
+ ]
+ },
+
+ // node.js
+ {
+ target: '**/vs/**/node/**',
+ allowedTypes: [
+ ...CORE_TYPES,
+
+ // --> types from node.d.ts that duplicate from lib.dom.d.ts
+ 'URL',
+ 'protocol',
+ 'hostname',
+ 'port',
+ 'pathname',
+ 'search',
+ 'username',
+ 'password'
+ ],
+ disallowedDefinitions: [
+ 'lib.dom.d.ts' // no DOM
+ ]
+ },
+
+ // Electron (sandbox)
+ {
+ target: '**/vs/**/electron-sandbox/**',
+ allowedTypes: CORE_TYPES,
+ disallowedDefinitions: [
+ '@types/node' // no node.js
+ ]
+ },
+
+ // Electron (renderer): skip
+ {
+ target: '**/vs/**/electron-browser/**',
+ skip: true // -> supports all types
+ },
+
+ // Electron (main)
+ {
+ target: '**/vs/**/electron-main/**',
+ allowedTypes: [
+ ...CORE_TYPES,
+
+ // --> types from electron.d.ts that duplicate from lib.dom.d.ts
+ 'Event',
+ 'Request'
+ ],
+ disallowedDefinitions: [
+ 'lib.dom.d.ts' // no DOM
+ ]
+ }
+];
+
+const TS_CONFIG_PATH = join(__dirname, '../../', 'src', 'tsconfig.json');
+
+interface IRule {
+ target: string;
+ skip?: boolean;
+ allowedTypes?: string[];
+ disallowedDefinitions?: string[];
+ disallowedTypes?: string[];
+}
+
+let hasErrors = false;
+
+function checkFile(program: ts.Program, sourceFile: ts.SourceFile, rule: IRule) {
+ checkNode(sourceFile);
+
+ function checkNode(node: ts.Node): void {
+ if (node.kind !== ts.SyntaxKind.Identifier) {
+ return ts.forEachChild(node, checkNode); // recurse down
+ }
+
+ const text = node.getText(sourceFile);
+
+ if (rule.allowedTypes?.some(allowed => allowed === text)) {
+ return; // override
+ }
+
+ if (rule.disallowedTypes?.some(disallowed => disallowed === text)) {
+ const { line, character } = sourceFile.getLineAndCharacterOfPosition(node.getStart());
+ console.log(`[build/lib/layersChecker.ts]: Reference to '${text}' violates layer '${rule.target}' (${sourceFile.fileName} (${line + 1},${character + 1})`);
+
+ hasErrors = true;
+ return;
+ }
+
+ const checker = program.getTypeChecker();
+ const symbol = checker.getSymbolAtLocation(node);
+ if (symbol) {
+ const declarations = symbol.declarations;
+ if (Array.isArray(declarations)) {
+ for (const declaration of declarations) {
+ if (declaration) {
+ const parent = declaration.parent;
+ if (parent) {
+ const parentSourceFile = parent.getSourceFile();
+ if (parentSourceFile) {
+ const definitionFileName = parentSourceFile.fileName;
+ if (rule.disallowedDefinitions) {
+ for (const disallowedDefinition of rule.disallowedDefinitions) {
+ if (definitionFileName.indexOf(disallowedDefinition) >= 0) {
+ const { line, character } = sourceFile.getLineAndCharacterOfPosition(node.getStart());
+ console.log(`[build/lib/layersChecker.ts]: Reference to '${text}' from '${disallowedDefinition}' violates layer '${rule.target}' (${sourceFile.fileName} (${line + 1},${character + 1})`);
+
+ hasErrors = true;
+ return;
+ }
+ }
+ }
+ }
+ }
+ }
+ }
+ }
+ }
+ }
+}
+
+function createProgram(tsconfigPath: string): ts.Program {
+ const tsConfig = ts.readConfigFile(tsconfigPath, ts.sys.readFile);
+
+ const configHostParser: ts.ParseConfigHost = { fileExists: existsSync, readDirectory: ts.sys.readDirectory, readFile: file => readFileSync(file, 'utf8'), useCaseSensitiveFileNames: process.platform === 'linux' };
+ const tsConfigParsed = ts.parseJsonConfigFileContent(tsConfig.config, configHostParser, resolve(dirname(tsconfigPath)), { noEmit: true });
+
+ const compilerHost = ts.createCompilerHost(tsConfigParsed.options, true);
+
+ return ts.createProgram(tsConfigParsed.fileNames, tsConfigParsed.options, compilerHost);
+}
+
+//
+// Create program and start checking
+//
+const program = createProgram(TS_CONFIG_PATH);
+
+for (const sourceFile of program.getSourceFiles()) {
+ for (const rule of RULES) {
+ if (match([sourceFile.fileName], rule.target).length > 0) {
+ if (!rule.skip) {
+ checkFile(program, sourceFile, rule);
+ }
+
+ break;
+ }
+ }
+}
+
+if (hasErrors) {
+ process.exit(1);
+}
diff --git a/build/lib/optimize.js b/build/lib/optimize.js
index 45f1169846301..5403c105620a1 100644
--- a/build/lib/optimize.js
+++ b/build/lib/optimize.js
@@ -4,8 +4,8 @@
*--------------------------------------------------------------------------------------------*/
'use strict';
Object.defineProperty(exports, "__esModule", { value: true });
+exports.minifyTask = exports.optimizeTask = exports.loaderConfig = void 0;
const es = require("event-stream");
-const fs = require("fs");
const gulp = require("gulp");
const concat = require("gulp-concat");
const minifyCSS = require("gulp-cssnano");
@@ -28,13 +28,13 @@ const REPO_ROOT_PATH = path.join(__dirname, '../..');
function log(prefix, message) {
fancyLog(ansiColors.cyan('[' + prefix + ']'), message);
}
-function loaderConfig(emptyPaths) {
+function loaderConfig() {
const result = {
paths: {
'vs': 'out-build/vs',
'vscode': 'empty:'
},
- nodeModules: emptyPaths || []
+ amdModulesPattern: /^vs\//
};
result['vs/css'] = { inlineResources: true };
return result;
@@ -68,14 +68,9 @@ function loader(src, bundledFileHeader, bundleLoader) {
this.emit('data', data);
}
}))
- .pipe(util.loadSourcemaps())
- .pipe(concat('vs/loader.js'))
- .pipe(es.mapSync(function (f) {
- f.sourceMap.sourceRoot = util.toFileUri(path.join(REPO_ROOT_PATH, 'src'));
- return f;
- })));
+ .pipe(concat('vs/loader.js')));
}
-function toConcatStream(src, bundledFileHeader, sources, dest) {
+function toConcatStream(src, bundledFileHeader, sources, dest, fileContentMapper) {
const useSourcemaps = /\.js$/.test(dest) && !/\.nls\.js$/.test(dest);
// If a bundle ends up including in any of the sources our copyright, then
// insert a fake source at the beginning of each bundle with our copyright
@@ -96,10 +91,12 @@ function toConcatStream(src, bundledFileHeader, sources, dest) {
const treatedSources = sources.map(function (source) {
const root = source.path ? REPO_ROOT_PATH.replace(/\\/g, '/') : '';
const base = source.path ? root + `/${src}` : '';
+ const path = source.path ? root + '/' + source.path.replace(/\\/g, '/') : 'fake';
+ const contents = source.path ? fileContentMapper(source.contents, path) : source.contents;
return new VinylFile({
- path: source.path ? root + '/' + source.path.replace(/\\/g, '/') : 'fake',
+ path: path,
base: base,
- contents: Buffer.from(source.contents)
+ contents: Buffer.from(contents)
});
});
return es.readArray(treatedSources)
@@ -107,9 +104,9 @@ function toConcatStream(src, bundledFileHeader, sources, dest) {
.pipe(concat(dest))
.pipe(stats_1.createStatsStream(dest));
}
-function toBundleStream(src, bundledFileHeader, bundles) {
+function toBundleStream(src, bundledFileHeader, bundles, fileContentMapper) {
return es.merge(bundles.map(function (bundle) {
- return toConcatStream(src, bundledFileHeader, bundle.sources, bundle.dest);
+ return toConcatStream(src, bundledFileHeader, bundle.sources, bundle.dest, fileContentMapper);
}));
}
const DEFAULT_FILE_HEADER = [
@@ -125,6 +122,7 @@ function optimizeTask(opts) {
const bundledFileHeader = opts.header || DEFAULT_FILE_HEADER;
const bundleLoader = (typeof opts.bundleLoader === 'undefined' ? true : opts.bundleLoader);
const out = opts.out;
+ const fileContentMapper = opts.fileContentMapper || ((contents, _path) => contents);
return function () {
const bundlesStream = es.through(); // this stream will contain the bundled files
const resourcesStream = es.through(); // this stream will contain the resources
@@ -133,15 +131,7 @@ function optimizeTask(opts) {
if (err || !result) {
return bundlesStream.emit('error', JSON.stringify(err));
}
- if (opts.inlineAmdImages) {
- try {
- result = inlineAmdImages(src, result);
- }
- catch (err) {
- return bundlesStream.emit('error', JSON.stringify(err));
- }
- }
- toBundleStream(src, bundledFileHeader, result.files).pipe(bundlesStream);
+ toBundleStream(src, bundledFileHeader, result.files, fileContentMapper).pipe(bundlesStream);
// Remove css inlined resources
const filteredResources = resources.slice();
result.cssInlinedResources.forEach(function (resource) {
@@ -176,39 +166,6 @@ function optimizeTask(opts) {
};
}
exports.optimizeTask = optimizeTask;
-function inlineAmdImages(src, result) {
- for (const outputFile of result.files) {
- for (const sourceFile of outputFile.sources) {
- if (sourceFile.path && /\.js$/.test(sourceFile.path)) {
- sourceFile.contents = sourceFile.contents.replace(/\([^.]+\.registerAndGetAmdImageURL\(([^)]+)\)\)/g, (_, m0) => {
- let imagePath = m0;
- // remove `` or ''
- if ((imagePath.charAt(0) === '`' && imagePath.charAt(imagePath.length - 1) === '`')
- || (imagePath.charAt(0) === '\'' && imagePath.charAt(imagePath.length - 1) === '\'')) {
- imagePath = imagePath.substr(1, imagePath.length - 2);
- }
- if (!/\.(png|svg)$/.test(imagePath)) {
- console.log(`original: ${_}`);
- return _;
- }
- const repoLocation = path.join(src, imagePath);
- const absoluteLocation = path.join(REPO_ROOT_PATH, repoLocation);
- if (!fs.existsSync(absoluteLocation)) {
- const message = `Invalid amd image url in file ${sourceFile.path}: ${imagePath}`;
- console.log(message);
- throw new Error(message);
- }
- const fileContents = fs.readFileSync(absoluteLocation);
- const mime = /\.svg$/.test(imagePath) ? 'image/svg+xml' : 'image/png';
- // Mark the file as inlined so we don't ship it by itself
- result.cssInlinedResources.push(repoLocation);
- return `("data:${mime};base64,${fileContents.toString('base64')}")`;
- });
- }
- }
- }
- return result;
-}
/**
* Wrap around uglify and allow the preserveComments function
* to have a file "context" to include our copyright only once per file.
diff --git a/build/lib/optimize.ts b/build/lib/optimize.ts
index 46189514c30a1..2822803f9f7a0 100644
--- a/build/lib/optimize.ts
+++ b/build/lib/optimize.ts
@@ -6,7 +6,6 @@
'use strict';
import * as es from 'event-stream';
-import * as fs from 'fs';
import * as gulp from 'gulp';
import * as concat from 'gulp-concat';
import * as minifyCSS from 'gulp-cssnano';
@@ -19,7 +18,6 @@ import * as fancyLog from 'fancy-log';
import * as ansiColors from 'ansi-colors';
import * as path from 'path';
import * as pump from 'pump';
-import * as sm from 'source-map';
import * as terser from 'terser';
import * as VinylFile from 'vinyl';
import * as bundle from './bundle';
@@ -33,13 +31,13 @@ function log(prefix: string, message: string): void {
fancyLog(ansiColors.cyan('[' + prefix + ']'), message);
}
-export function loaderConfig(emptyPaths?: string[]) {
+export function loaderConfig() {
const result: any = {
paths: {
'vs': 'out-build/vs',
'vscode': 'empty:'
},
- nodeModules: emptyPaths || []
+ amdModulesPattern: /^vs\//
};
result['vs/css'] = { inlineResources: true };
@@ -49,10 +47,6 @@ export function loaderConfig(emptyPaths?: string[]) {
const IS_OUR_COPYRIGHT_REGEXP = /Copyright \(C\) Microsoft Corporation/i;
-declare class FileSourceMap extends VinylFile {
- public sourceMap: sm.RawSourceMap;
-}
-
function loader(src: string, bundledFileHeader: string, bundleLoader: boolean): NodeJS.ReadWriteStream {
let sources = [
`${src}/vs/loader.js`
@@ -81,16 +75,11 @@ function loader(src: string, bundledFileHeader: string, bundleLoader: boolean):
this.emit('data', data);
}
}))
- .pipe(util.loadSourcemaps())
.pipe(concat('vs/loader.js'))
- .pipe(es.mapSync(function (f) {
- f.sourceMap.sourceRoot = util.toFileUri(path.join(REPO_ROOT_PATH, 'src'));
- return f;
- }))
);
}
-function toConcatStream(src: string, bundledFileHeader: string, sources: bundle.IFile[], dest: string): NodeJS.ReadWriteStream {
+function toConcatStream(src: string, bundledFileHeader: string, sources: bundle.IFile[], dest: string, fileContentMapper: (contents: string, path: string) => string): NodeJS.ReadWriteStream {
const useSourcemaps = /\.js$/.test(dest) && !/\.nls\.js$/.test(dest);
// If a bundle ends up including in any of the sources our copyright, then
@@ -114,11 +103,13 @@ function toConcatStream(src: string, bundledFileHeader: string, sources: bundle.
const treatedSources = sources.map(function (source) {
const root = source.path ? REPO_ROOT_PATH.replace(/\\/g, '/') : '';
const base = source.path ? root + `/${src}` : '';
+ const path = source.path ? root + '/' + source.path.replace(/\\/g, '/') : 'fake';
+ const contents = source.path ? fileContentMapper(source.contents, path) : source.contents;
return new VinylFile({
- path: source.path ? root + '/' + source.path.replace(/\\/g, '/') : 'fake',
+ path: path,
base: base,
- contents: Buffer.from(source.contents)
+ contents: Buffer.from(contents)
});
});
@@ -128,9 +119,9 @@ function toConcatStream(src: string, bundledFileHeader: string, sources: bundle.
.pipe(createStatsStream(dest));
}
-function toBundleStream(src: string, bundledFileHeader: string, bundles: bundle.IConcatFile[]): NodeJS.ReadWriteStream {
+function toBundleStream(src: string, bundledFileHeader: string, bundles: bundle.IConcatFile[], fileContentMapper: (contents: string, path: string) => string): NodeJS.ReadWriteStream {
return es.merge(bundles.map(function (bundle) {
- return toConcatStream(src, bundledFileHeader, bundle.sources, bundle.dest);
+ return toConcatStream(src, bundledFileHeader, bundle.sources, bundle.dest, fileContentMapper);
}));
}
@@ -160,10 +151,6 @@ export interface IOptimizeTaskOpts {
* (emit bundleInfo.json file)
*/
bundleInfo: boolean;
- /**
- * replace calls to `registerAndGetAmdImageURL` with data uris
- */
- inlineAmdImages: boolean;
/**
* (out folder name)
*/
@@ -172,6 +159,12 @@ export interface IOptimizeTaskOpts {
* (out folder name)
*/
languages?: Language[];
+ /**
+ * File contents interceptor
+ * @param contents The contens of the file
+ * @param path The absolute file path, always using `/`, even on Windows
+ */
+ fileContentMapper?: (contents: string, path: string) => string;
}
const DEFAULT_FILE_HEADER = [
@@ -188,6 +181,7 @@ export function optimizeTask(opts: IOptimizeTaskOpts): () => NodeJS.ReadWriteStr
const bundledFileHeader = opts.header || DEFAULT_FILE_HEADER;
const bundleLoader = (typeof opts.bundleLoader === 'undefined' ? true : opts.bundleLoader);
const out = opts.out;
+ const fileContentMapper = opts.fileContentMapper || ((contents: string, _path: string) => contents);
return function () {
const bundlesStream = es.through(); // this stream will contain the bundled files
@@ -197,15 +191,7 @@ export function optimizeTask(opts: IOptimizeTaskOpts): () => NodeJS.ReadWriteStr
bundle.bundle(entryPoints, loaderConfig, function (err, result) {
if (err || !result) { return bundlesStream.emit('error', JSON.stringify(err)); }
- if (opts.inlineAmdImages) {
- try {
- result = inlineAmdImages(src, result);
- } catch (err) {
- return bundlesStream.emit('error', JSON.stringify(err));
- }
- }
-
- toBundleStream(src, bundledFileHeader, result.files).pipe(bundlesStream);
+ toBundleStream(src, bundledFileHeader, result.files, fileContentMapper).pipe(bundlesStream);
// Remove css inlined resources
const filteredResources = resources.slice();
@@ -249,42 +235,6 @@ export function optimizeTask(opts: IOptimizeTaskOpts): () => NodeJS.ReadWriteStr
};
}
-function inlineAmdImages(src: string, result: bundle.IBundleResult): bundle.IBundleResult {
- for (const outputFile of result.files) {
- for (const sourceFile of outputFile.sources) {
- if (sourceFile.path && /\.js$/.test(sourceFile.path)) {
- sourceFile.contents = sourceFile.contents.replace(/\([^.]+\.registerAndGetAmdImageURL\(([^)]+)\)\)/g, (_, m0) => {
- let imagePath = m0;
- // remove `` or ''
- if ((imagePath.charAt(0) === '`' && imagePath.charAt(imagePath.length - 1) === '`')
- || (imagePath.charAt(0) === '\'' && imagePath.charAt(imagePath.length - 1) === '\'')) {
- imagePath = imagePath.substr(1, imagePath.length - 2);
- }
- if (!/\.(png|svg)$/.test(imagePath)) {
- console.log(`original: ${_}`);
- return _;
- }
- const repoLocation = path.join(src, imagePath);
- const absoluteLocation = path.join(REPO_ROOT_PATH, repoLocation);
- if (!fs.existsSync(absoluteLocation)) {
- const message = `Invalid amd image url in file ${sourceFile.path}: ${imagePath}`;
- console.log(message);
- throw new Error(message);
- }
- const fileContents = fs.readFileSync(absoluteLocation);
- const mime = /\.svg$/.test(imagePath) ? 'image/svg+xml' : 'image/png';
-
- // Mark the file as inlined so we don't ship it by itself
- result.cssInlinedResources.push(repoLocation);
-
- return `("data:${mime};base64,${fileContents.toString('base64')}")`;
- });
- }
- }
- }
- return result;
-}
-
declare class FileWithCopyright extends VinylFile {
public __hasOurCopyright: boolean;
}
diff --git a/build/lib/preLaunch.js b/build/lib/preLaunch.js
new file mode 100644
index 0000000000000..1aecbe190487e
--- /dev/null
+++ b/build/lib/preLaunch.js
@@ -0,0 +1,55 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+'use strict';
+Object.defineProperty(exports, "__esModule", { value: true });
+// @ts-check
+const path = require("path");
+const child_process_1 = require("child_process");
+const fs_1 = require("fs");
+const yarn = process.platform === 'win32' ? 'yarn.cmd' : 'yarn';
+const rootDir = path.resolve(__dirname, '..', '..');
+function runProcess(command, args = []) {
+ return new Promise((resolve, reject) => {
+ const child = child_process_1.spawn(command, args, { cwd: rootDir, stdio: 'inherit', env: process.env });
+ child.on('exit', err => !err ? resolve() : process.exit(err !== null && err !== void 0 ? err : 1));
+ child.on('error', reject);
+ });
+}
+async function exists(subdir) {
+ try {
+ await fs_1.promises.stat(path.join(rootDir, subdir));
+ return true;
+ }
+ catch (_a) {
+ return false;
+ }
+}
+async function ensureNodeModules() {
+ if (!(await exists('node_modules'))) {
+ await runProcess(yarn);
+ }
+}
+async function getElectron() {
+ await runProcess(yarn, ['electron']);
+}
+async function ensureCompiled() {
+ if (!(await exists('out'))) {
+ await runProcess(yarn, ['compile']);
+ }
+}
+async function main() {
+ await ensureNodeModules();
+ await getElectron();
+ await ensureCompiled();
+ // Can't require this until after dependencies are installed
+ const { getBuiltInExtensions } = require('./builtInExtensions');
+ await getBuiltInExtensions();
+}
+if (require.main === module) {
+ main().catch(err => {
+ console.error(err);
+ process.exit(1);
+ });
+}
diff --git a/build/lib/preLaunch.ts b/build/lib/preLaunch.ts
new file mode 100644
index 0000000000000..57441870cc4d9
--- /dev/null
+++ b/build/lib/preLaunch.ts
@@ -0,0 +1,65 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+'use strict';
+
+// @ts-check
+
+import * as path from 'path';
+import { spawn } from 'child_process';
+import { promises as fs } from 'fs';
+
+const yarn = process.platform === 'win32' ? 'yarn.cmd' : 'yarn';
+const rootDir = path.resolve(__dirname, '..', '..');
+
+function runProcess(command: string, args: ReadonlyArray = []) {
+ return new Promise((resolve, reject) => {
+ const child = spawn(command, args, { cwd: rootDir, stdio: 'inherit', env: process.env });
+ child.on('exit', err => !err ? resolve() : process.exit(err ?? 1));
+ child.on('error', reject);
+ });
+}
+
+async function exists(subdir: string) {
+ try {
+ await fs.stat(path.join(rootDir, subdir));
+ return true;
+ } catch {
+ return false;
+ }
+}
+
+async function ensureNodeModules() {
+ if (!(await exists('node_modules'))) {
+ await runProcess(yarn);
+ }
+}
+
+async function getElectron() {
+ await runProcess(yarn, ['electron']);
+}
+
+async function ensureCompiled() {
+ if (!(await exists('out'))) {
+ await runProcess(yarn, ['compile']);
+ }
+}
+
+async function main() {
+ await ensureNodeModules();
+ await getElectron();
+ await ensureCompiled();
+
+ // Can't require this until after dependencies are installed
+ const { getBuiltInExtensions } = require('./builtInExtensions');
+ await getBuiltInExtensions();
+}
+
+if (require.main === module) {
+ main().catch(err => {
+ console.error(err);
+ process.exit(1);
+ });
+}
diff --git a/build/lib/reporter.js b/build/lib/reporter.js
index e0461dc6d9d38..67615bf48dc46 100644
--- a/build/lib/reporter.js
+++ b/build/lib/reporter.js
@@ -4,6 +4,7 @@
*--------------------------------------------------------------------------------------------*/
'use strict';
Object.defineProperty(exports, "__esModule", { value: true });
+exports.createReporter = void 0;
const es = require("event-stream");
const _ = require("underscore");
const fancyLog = require("fancy-log");
diff --git a/build/lib/standalone.js b/build/lib/standalone.js
index 56f89544dac98..531194c35fdcd 100644
--- a/build/lib/standalone.js
+++ b/build/lib/standalone.js
@@ -4,6 +4,7 @@
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
Object.defineProperty(exports, "__esModule", { value: true });
+exports.createESMSourcesAndResources2 = exports.extractEditor = void 0;
const ts = require("typescript");
const fs = require("fs");
const path = require("path");
@@ -43,7 +44,9 @@ function extractEditor(options) {
compilerOptions.declaration = false;
compilerOptions.moduleResolution = ts.ModuleResolutionKind.Classic;
options.compilerOptions = compilerOptions;
- console.log(`Running with shakeLevel ${tss.toStringShakeLevel(options.shakeLevel)}`);
+ console.log(`Running tree shaker with shakeLevel ${tss.toStringShakeLevel(options.shakeLevel)}`);
+ // Take the extra included .d.ts files from `tsconfig.monaco.json`
+ options.typings = tsConfig.include.filter(includedFile => /\.d\.ts$/.test(includedFile));
let result = tss.shake(options);
for (let fileName in result) {
if (result.hasOwnProperty(fileName)) {
diff --git a/build/lib/standalone.ts b/build/lib/standalone.ts
index 07000331ad2b6..f80c611e6fc7b 100644
--- a/build/lib/standalone.ts
+++ b/build/lib/standalone.ts
@@ -50,7 +50,10 @@ export function extractEditor(options: tss.ITreeShakingOptions & { destRoot: str
options.compilerOptions = compilerOptions;
- console.log(`Running with shakeLevel ${tss.toStringShakeLevel(options.shakeLevel)}`);
+ console.log(`Running tree shaker with shakeLevel ${tss.toStringShakeLevel(options.shakeLevel)}`);
+
+ // Take the extra included .d.ts files from `tsconfig.monaco.json`
+ options.typings = (tsConfig.include).filter(includedFile => /\.d\.ts$/.test(includedFile));
let result = tss.shake(options);
for (let fileName in result) {
diff --git a/build/lib/stats.js b/build/lib/stats.js
index 99ad665f22320..2ff02e405a68a 100644
--- a/build/lib/stats.js
+++ b/build/lib/stats.js
@@ -4,6 +4,7 @@
*--------------------------------------------------------------------------------------------*/
'use strict';
Object.defineProperty(exports, "__esModule", { value: true });
+exports.submitAllStats = exports.createStatsStream = void 0;
const es = require("event-stream");
const fancyLog = require("fancy-log");
const ansiColors = require("ansi-colors");
diff --git a/build/lib/task.js b/build/lib/task.js
index f1e6e3f6245de..d08ab8acde8f0 100644
--- a/build/lib/task.js
+++ b/build/lib/task.js
@@ -4,6 +4,7 @@
*--------------------------------------------------------------------------------------------*/
'use strict';
Object.defineProperty(exports, "__esModule", { value: true });
+exports.define = exports.parallel = exports.series = void 0;
const fancyLog = require("fancy-log");
const ansiColors = require("ansi-colors");
function _isPromise(p) {
diff --git a/build/lib/treeshaking.js b/build/lib/treeshaking.js
index 50ff7caa01d3b..5b1cf0591eca9 100644
--- a/build/lib/treeshaking.js
+++ b/build/lib/treeshaking.js
@@ -4,6 +4,7 @@
*--------------------------------------------------------------------------------------------*/
'use strict';
Object.defineProperty(exports, "__esModule", { value: true });
+exports.shake = exports.toStringShakeLevel = exports.ShakeLevel = void 0;
const fs = require("fs");
const path = require("path");
const ts = require("typescript");
@@ -25,17 +26,17 @@ function toStringShakeLevel(shakeLevel) {
}
}
exports.toStringShakeLevel = toStringShakeLevel;
-function printDiagnostics(diagnostics) {
+function printDiagnostics(options, diagnostics) {
for (const diag of diagnostics) {
let result = '';
if (diag.file) {
- result += `${diag.file.fileName}: `;
+ result += `${path.join(options.sourcesRoot, diag.file.fileName)}`;
}
if (diag.file && diag.start) {
let location = diag.file.getLineAndCharacterOfPosition(diag.start);
- result += `- ${location.line + 1},${location.character} - `;
+ result += `:${location.line + 1}:${location.character}`;
}
- result += JSON.stringify(diag.messageText);
+ result += ` - ` + JSON.stringify(diag.messageText);
console.log(result);
}
}
@@ -44,17 +45,17 @@ function shake(options) {
const program = languageService.getProgram();
const globalDiagnostics = program.getGlobalDiagnostics();
if (globalDiagnostics.length > 0) {
- printDiagnostics(globalDiagnostics);
+ printDiagnostics(options, globalDiagnostics);
throw new Error(`Compilation Errors encountered.`);
}
const syntacticDiagnostics = program.getSyntacticDiagnostics();
if (syntacticDiagnostics.length > 0) {
- printDiagnostics(syntacticDiagnostics);
+ printDiagnostics(options, syntacticDiagnostics);
throw new Error(`Compilation Errors encountered.`);
}
const semanticDiagnostics = program.getSemanticDiagnostics();
if (semanticDiagnostics.length > 0) {
- printDiagnostics(semanticDiagnostics);
+ printDiagnostics(options, semanticDiagnostics);
throw new Error(`Compilation Errors encountered.`);
}
markNodes(languageService, options);
@@ -75,11 +76,7 @@ function createTypeScriptLanguageService(options) {
FILES[typing] = fs.readFileSync(filePath).toString();
});
// Resolve libs
- const RESOLVED_LIBS = {};
- options.libs.forEach((filename) => {
- const filepath = path.join(TYPESCRIPT_LIB_FOLDER, filename);
- RESOLVED_LIBS[`defaultLib:${filename}`] = fs.readFileSync(filepath).toString();
- });
+ const RESOLVED_LIBS = processLibFiles(options);
const compilerOptions = ts.convertCompilerOptionsFromJson(options.compilerOptions, options.sourcesRoot).options;
const host = new TypeScriptLanguageServiceHost(RESOLVED_LIBS, FILES, compilerOptions);
return ts.createLanguageService(host);
@@ -137,6 +134,29 @@ function discoverAndReadFiles(options) {
}
return FILES;
}
+/**
+ * Read lib files and follow lib references
+ */
+function processLibFiles(options) {
+ const stack = [...options.compilerOptions.lib];
+ const result = {};
+ while (stack.length > 0) {
+ const filename = `lib.${stack.shift().toLowerCase()}.d.ts`;
+ const key = `defaultLib:${filename}`;
+ if (!result[key]) {
+ // add this file
+ const filepath = path.join(TYPESCRIPT_LIB_FOLDER, filename);
+ const sourceText = fs.readFileSync(filepath).toString();
+ result[key] = sourceText;
+ // precess dependencies and "recurse"
+ const info = ts.preProcessFile(sourceText);
+ for (let ref of info.libReferenceDirectives) {
+ stack.push(ref.fileName);
+ }
+ }
+ }
+ return result;
+}
/**
* A TypeScript language service host
*/
@@ -234,6 +254,7 @@ function markNodes(languageService, options) {
}
const black_queue = [];
const gray_queue = [];
+ const export_import_queue = [];
const sourceFilesLoaded = {};
function enqueueTopLevelModuleStatements(sourceFile) {
sourceFile.forEachChild((node) => {
@@ -245,10 +266,16 @@ function markNodes(languageService, options) {
return;
}
if (ts.isExportDeclaration(node)) {
- if (node.moduleSpecifier && ts.isStringLiteral(node.moduleSpecifier)) {
+ if (!node.exportClause && node.moduleSpecifier && ts.isStringLiteral(node.moduleSpecifier)) {
+ // export * from "foo";
setColor(node, 2 /* Black */);
enqueueImport(node, node.moduleSpecifier.text);
}
+ if (node.exportClause && ts.isNamedExports(node.exportClause)) {
+ for (const exportSpecifier of node.exportClause.elements) {
+ export_import_queue.push(exportSpecifier);
+ }
+ }
return;
}
if (ts.isExpressionStatement(node)
@@ -306,7 +333,7 @@ function markNodes(languageService, options) {
}
setColor(node, 2 /* Black */);
black_queue.push(node);
- if (options.shakeLevel === 2 /* ClassMembers */ && (ts.isMethodDeclaration(node) || ts.isMethodSignature(node) || ts.isPropertySignature(node) || ts.isGetAccessor(node) || ts.isSetAccessor(node))) {
+ if (options.shakeLevel === 2 /* ClassMembers */ && (ts.isMethodDeclaration(node) || ts.isMethodSignature(node) || ts.isPropertySignature(node) || ts.isPropertyDeclaration(node) || ts.isGetAccessor(node) || ts.isSetAccessor(node))) {
const references = languageService.getReferencesAtPosition(node.getSourceFile().fileName, node.name.pos + node.name.getLeadingTriviaWidth());
if (references) {
for (let i = 0, len = references.length; i < len; i++) {
@@ -358,7 +385,7 @@ function markNodes(languageService, options) {
++step;
let node;
if (step % 100 === 0) {
- console.log(`${step}/${step + black_queue.length + gray_queue.length} (${black_queue.length}, ${gray_queue.length})`);
+ console.log(`Treeshaking - ${Math.floor(100 * step / (step + black_queue.length + gray_queue.length))}% - ${step}/${step + black_queue.length + gray_queue.length} (${black_queue.length}, ${gray_queue.length})`);
}
if (black_queue.length === 0) {
for (let i = 0; i < gray_queue.length; i++) {
@@ -393,7 +420,7 @@ function markNodes(languageService, options) {
// (they can be the declaration of a module import)
continue;
}
- if (options.shakeLevel === 2 /* ClassMembers */ && (ts.isClassDeclaration(declaration) || ts.isInterfaceDeclaration(declaration))) {
+ if (options.shakeLevel === 2 /* ClassMembers */ && (ts.isClassDeclaration(declaration) || ts.isInterfaceDeclaration(declaration)) && !isLocalCodeExtendingOrInheritingFromDefaultLibSymbol(program, checker, declaration)) {
enqueue_black(declaration.name);
for (let j = 0; j < declaration.members.length; j++) {
const member = declaration.members[j];
@@ -402,6 +429,8 @@ function markNodes(languageService, options) {
|| ts.isConstructSignatureDeclaration(member)
|| ts.isIndexSignatureDeclaration(member)
|| ts.isCallSignatureDeclaration(member)
+ || memberName === '[Symbol.iterator]'
+ || memberName === '[Symbol.toStringTag]'
|| memberName === 'toJSON'
|| memberName === 'toString'
|| memberName === 'dispose' // TODO: keeping all `dispose` methods
@@ -426,6 +455,22 @@ function markNodes(languageService, options) {
};
node.forEachChild(loop);
}
+ while (export_import_queue.length > 0) {
+ const node = export_import_queue.shift();
+ if (nodeOrParentIsBlack(node)) {
+ continue;
+ }
+ const symbol = node.symbol;
+ if (!symbol) {
+ continue;
+ }
+ const aliased = checker.getAliasedSymbol(symbol);
+ if (aliased.declarations && aliased.declarations.length > 0) {
+ if (nodeOrParentIsBlack(aliased.declarations[0]) || nodeOrChildIsBlack(aliased.declarations[0])) {
+ setColor(node, 2 /* Black */);
+ }
+ }
+ }
}
function nodeIsInItsOwnDeclaration(nodeSourceFile, node, symbol) {
for (let i = 0, len = symbol.declarations.length; i < len; i++) {
@@ -517,6 +562,21 @@ function generateResult(languageService, shakeLevel) {
}
}
}
+ if (ts.isExportDeclaration(node)) {
+ if (node.exportClause && node.moduleSpecifier && ts.isNamedExports(node.exportClause)) {
+ let survivingExports = [];
+ for (const exportSpecifier of node.exportClause.elements) {
+ if (getColor(exportSpecifier) === 2 /* Black */) {
+ survivingExports.push(exportSpecifier.getFullText(sourceFile));
+ }
+ }
+ const leadingTriviaWidth = node.getLeadingTriviaWidth();
+ const leadingTrivia = sourceFile.text.substr(node.pos, leadingTriviaWidth);
+ if (survivingExports.length > 0) {
+ return write(`${leadingTrivia}export {${survivingExports.join(',')} } from${node.moduleSpecifier.getFullText(sourceFile)};`);
+ }
+ }
+ }
if (shakeLevel === 2 /* ClassMembers */ && (ts.isClassDeclaration(node) || ts.isInterfaceDeclaration(node)) && nodeOrChildIsBlack(node)) {
let toWrite = node.getFullText();
for (let i = node.members.length - 1; i >= 0; i--) {
@@ -554,6 +614,34 @@ function generateResult(languageService, shakeLevel) {
}
//#endregion
//#region Utils
+function isLocalCodeExtendingOrInheritingFromDefaultLibSymbol(program, checker, declaration) {
+ if (!program.isSourceFileDefaultLibrary(declaration.getSourceFile()) && declaration.heritageClauses) {
+ for (const heritageClause of declaration.heritageClauses) {
+ for (const type of heritageClause.types) {
+ const symbol = findSymbolFromHeritageType(checker, type);
+ if (symbol) {
+ const decl = symbol.valueDeclaration || (symbol.declarations && symbol.declarations[0]);
+ if (decl && program.isSourceFileDefaultLibrary(decl.getSourceFile())) {
+ return true;
+ }
+ }
+ }
+ }
+ }
+ return false;
+}
+function findSymbolFromHeritageType(checker, type) {
+ if (ts.isExpressionWithTypeArguments(type)) {
+ return findSymbolFromHeritageType(checker, type.expression);
+ }
+ if (ts.isIdentifier(type)) {
+ return getRealNodeSymbol(checker, type)[0];
+ }
+ if (ts.isPropertyAccessExpression(type)) {
+ return findSymbolFromHeritageType(checker, type.name);
+ }
+ return null;
+}
/**
* Returns the node's symbol and the `import` node (if the symbol resolved from a different module)
*/
@@ -567,7 +655,7 @@ function getRealNodeSymbol(checker, node) {
// (2) when the aliased symbol is originating from an import.
//
function shouldSkipAlias(node, declaration) {
- if (node.kind !== ts.SyntaxKind.Identifier) {
+ if (!ts.isShorthandPropertyAssignment(node) && node.kind !== ts.SyntaxKind.Identifier) {
return false;
}
if (node.parent === declaration) {
@@ -589,7 +677,9 @@ function getRealNodeSymbol(checker, node) {
}
}
const { parent } = node;
- let symbol = checker.getSymbolAtLocation(node);
+ let symbol = (ts.isShorthandPropertyAssignment(node)
+ ? checker.getShorthandAssignmentValueSymbol(node)
+ : checker.getSymbolAtLocation(node));
let importNode = null;
// If this is an alias, and the request came at the declaration location
// get the aliased symbol instead. This allows for goto def on an import e.g.
diff --git a/build/lib/treeshaking.ts b/build/lib/treeshaking.ts
index f096963365582..405336bfcbb0a 100644
--- a/build/lib/treeshaking.ts
+++ b/build/lib/treeshaking.ts
@@ -18,7 +18,7 @@ export const enum ShakeLevel {
}
export function toStringShakeLevel(shakeLevel: ShakeLevel): string {
- switch(shakeLevel) {
+ switch (shakeLevel) {
case ShakeLevel.Files:
return 'Files (0)';
case ShakeLevel.InnerFile:
@@ -42,11 +42,6 @@ export interface ITreeShakingOptions {
* Inline usages.
*/
inlineEntryPoints: string[];
- /**
- * TypeScript libs.
- * e.g. `lib.d.ts`, `lib.es2015.collection.d.ts`
- */
- libs: string[];
/**
* Other .d.ts files
*/
@@ -71,17 +66,17 @@ export interface ITreeShakingResult {
[file: string]: string;
}
-function printDiagnostics(diagnostics: ReadonlyArray): void {
+function printDiagnostics(options: ITreeShakingOptions, diagnostics: ReadonlyArray): void {
for (const diag of diagnostics) {
let result = '';
if (diag.file) {
- result += `${diag.file.fileName}: `;
+ result += `${path.join(options.sourcesRoot, diag.file.fileName)}`;
}
if (diag.file && diag.start) {
let location = diag.file.getLineAndCharacterOfPosition(diag.start);
- result += `- ${location.line + 1},${location.character} - `;
+ result += `:${location.line + 1}:${location.character}`;
}
- result += JSON.stringify(diag.messageText);
+ result += ` - ` + JSON.stringify(diag.messageText);
console.log(result);
}
}
@@ -92,19 +87,19 @@ export function shake(options: ITreeShakingOptions): ITreeShakingResult {
const globalDiagnostics = program.getGlobalDiagnostics();
if (globalDiagnostics.length > 0) {
- printDiagnostics(globalDiagnostics);
+ printDiagnostics(options, globalDiagnostics);
throw new Error(`Compilation Errors encountered.`);
}
const syntacticDiagnostics = program.getSyntacticDiagnostics();
if (syntacticDiagnostics.length > 0) {
- printDiagnostics(syntacticDiagnostics);
+ printDiagnostics(options, syntacticDiagnostics);
throw new Error(`Compilation Errors encountered.`);
}
const semanticDiagnostics = program.getSemanticDiagnostics();
if (semanticDiagnostics.length > 0) {
- printDiagnostics(semanticDiagnostics);
+ printDiagnostics(options, semanticDiagnostics);
throw new Error(`Compilation Errors encountered.`);
}
@@ -130,11 +125,7 @@ function createTypeScriptLanguageService(options: ITreeShakingOptions): ts.Langu
});
// Resolve libs
- const RESOLVED_LIBS: ILibMap = {};
- options.libs.forEach((filename) => {
- const filepath = path.join(TYPESCRIPT_LIB_FOLDER, filename);
- RESOLVED_LIBS[`defaultLib:${filename}`] = fs.readFileSync(filepath).toString();
- });
+ const RESOLVED_LIBS = processLibFiles(options);
const compilerOptions = ts.convertCompilerOptionsFromJson(options.compilerOptions, options.sourcesRoot).options;
@@ -205,6 +196,34 @@ function discoverAndReadFiles(options: ITreeShakingOptions): IFileMap {
return FILES;
}
+/**
+ * Read lib files and follow lib references
+ */
+function processLibFiles(options: ITreeShakingOptions): ILibMap {
+
+ const stack: string[] = [...options.compilerOptions.lib];
+ const result: ILibMap = {};
+
+ while (stack.length > 0) {
+ const filename = `lib.${stack.shift()!.toLowerCase()}.d.ts`;
+ const key = `defaultLib:${filename}`;
+ if (!result[key]) {
+ // add this file
+ const filepath = path.join(TYPESCRIPT_LIB_FOLDER, filename);
+ const sourceText = fs.readFileSync(filepath).toString();
+ result[key] = sourceText;
+
+ // precess dependencies and "recurse"
+ const info = ts.preProcessFile(sourceText);
+ for (let ref of info.libReferenceDirectives) {
+ stack.push(ref.fileName);
+ }
+ }
+ }
+
+ return result;
+}
+
interface ILibMap { [libName: string]: string; }
interface IFileMap { [fileName: string]: string; }
@@ -317,6 +336,7 @@ function markNodes(languageService: ts.LanguageService, options: ITreeShakingOpt
const black_queue: ts.Node[] = [];
const gray_queue: ts.Node[] = [];
+ const export_import_queue: ts.Node[] = [];
const sourceFilesLoaded: { [fileName: string]: boolean } = {};
function enqueueTopLevelModuleStatements(sourceFile: ts.SourceFile): void {
@@ -332,10 +352,16 @@ function markNodes(languageService: ts.LanguageService, options: ITreeShakingOpt
}
if (ts.isExportDeclaration(node)) {
- if (node.moduleSpecifier && ts.isStringLiteral(node.moduleSpecifier)) {
+ if (!node.exportClause && node.moduleSpecifier && ts.isStringLiteral(node.moduleSpecifier)) {
+ // export * from "foo";
setColor(node, NodeColor.Black);
enqueueImport(node, node.moduleSpecifier.text);
}
+ if (node.exportClause && ts.isNamedExports(node.exportClause)) {
+ for (const exportSpecifier of node.exportClause.elements) {
+ export_import_queue.push(exportSpecifier);
+ }
+ }
return;
}
@@ -410,7 +436,7 @@ function markNodes(languageService: ts.LanguageService, options: ITreeShakingOpt
setColor(node, NodeColor.Black);
black_queue.push(node);
- if (options.shakeLevel === ShakeLevel.ClassMembers && (ts.isMethodDeclaration(node) || ts.isMethodSignature(node) || ts.isPropertySignature(node) || ts.isGetAccessor(node) || ts.isSetAccessor(node))) {
+ if (options.shakeLevel === ShakeLevel.ClassMembers && (ts.isMethodDeclaration(node) || ts.isMethodSignature(node) || ts.isPropertySignature(node) || ts.isPropertyDeclaration(node) || ts.isGetAccessor(node) || ts.isSetAccessor(node))) {
const references = languageService.getReferencesAtPosition(node.getSourceFile().fileName, node.name.pos + node.name.getLeadingTriviaWidth());
if (references) {
for (let i = 0, len = references.length; i < len; i++) {
@@ -471,11 +497,11 @@ function markNodes(languageService: ts.LanguageService, options: ITreeShakingOpt
let node: ts.Node;
if (step % 100 === 0) {
- console.log(`${step}/${step + black_queue.length + gray_queue.length} (${black_queue.length}, ${gray_queue.length})`);
+ console.log(`Treeshaking - ${Math.floor(100 * step / (step + black_queue.length + gray_queue.length))}% - ${step}/${step + black_queue.length + gray_queue.length} (${black_queue.length}, ${gray_queue.length})`);
}
if (black_queue.length === 0) {
- for (let i = 0; i< gray_queue.length; i++) {
+ for (let i = 0; i < gray_queue.length; i++) {
const node = gray_queue[i];
const nodeParent = node.parent;
if ((ts.isClassDeclaration(nodeParent) || ts.isInterfaceDeclaration(nodeParent)) && nodeOrChildIsBlack(nodeParent)) {
@@ -510,7 +536,7 @@ function markNodes(languageService: ts.LanguageService, options: ITreeShakingOpt
continue;
}
- if (options.shakeLevel === ShakeLevel.ClassMembers && (ts.isClassDeclaration(declaration) || ts.isInterfaceDeclaration(declaration))) {
+ if (options.shakeLevel === ShakeLevel.ClassMembers && (ts.isClassDeclaration(declaration) || ts.isInterfaceDeclaration(declaration)) && !isLocalCodeExtendingOrInheritingFromDefaultLibSymbol(program, checker, declaration)) {
enqueue_black(declaration.name!);
for (let j = 0; j < declaration.members.length; j++) {
@@ -521,6 +547,8 @@ function markNodes(languageService: ts.LanguageService, options: ITreeShakingOpt
|| ts.isConstructSignatureDeclaration(member)
|| ts.isIndexSignatureDeclaration(member)
|| ts.isCallSignatureDeclaration(member)
+ || memberName === '[Symbol.iterator]'
+ || memberName === '[Symbol.toStringTag]'
|| memberName === 'toJSON'
|| memberName === 'toString'
|| memberName === 'dispose'// TODO: keeping all `dispose` methods
@@ -545,6 +573,23 @@ function markNodes(languageService: ts.LanguageService, options: ITreeShakingOpt
};
node.forEachChild(loop);
}
+
+ while (export_import_queue.length > 0) {
+ const node = export_import_queue.shift()!;
+ if (nodeOrParentIsBlack(node)) {
+ continue;
+ }
+ const symbol: ts.Symbol | undefined = (node).symbol;
+ if (!symbol) {
+ continue;
+ }
+ const aliased = checker.getAliasedSymbol(symbol);
+ if (aliased.declarations && aliased.declarations.length > 0) {
+ if (nodeOrParentIsBlack(aliased.declarations[0]) || nodeOrChildIsBlack(aliased.declarations[0])) {
+ setColor(node, NodeColor.Black);
+ }
+ }
+ }
}
function nodeIsInItsOwnDeclaration(nodeSourceFile: ts.SourceFile, node: ts.Node, symbol: ts.Symbol): boolean {
@@ -646,6 +691,22 @@ function generateResult(languageService: ts.LanguageService, shakeLevel: ShakeLe
}
}
+ if (ts.isExportDeclaration(node)) {
+ if (node.exportClause && node.moduleSpecifier && ts.isNamedExports(node.exportClause)) {
+ let survivingExports: string[] = [];
+ for (const exportSpecifier of node.exportClause.elements) {
+ if (getColor(exportSpecifier) === NodeColor.Black) {
+ survivingExports.push(exportSpecifier.getFullText(sourceFile));
+ }
+ }
+ const leadingTriviaWidth = node.getLeadingTriviaWidth();
+ const leadingTrivia = sourceFile.text.substr(node.pos, leadingTriviaWidth);
+ if (survivingExports.length > 0) {
+ return write(`${leadingTrivia}export {${survivingExports.join(',')} } from${node.moduleSpecifier.getFullText(sourceFile)};`);
+ }
+ }
+ }
+
if (shakeLevel === ShakeLevel.ClassMembers && (ts.isClassDeclaration(node) || ts.isInterfaceDeclaration(node)) && nodeOrChildIsBlack(node)) {
let toWrite = node.getFullText();
for (let i = node.members.length - 1; i >= 0; i--) {
@@ -691,6 +752,36 @@ function generateResult(languageService: ts.LanguageService, shakeLevel: ShakeLe
//#region Utils
+function isLocalCodeExtendingOrInheritingFromDefaultLibSymbol(program: ts.Program, checker: ts.TypeChecker, declaration: ts.ClassDeclaration | ts.InterfaceDeclaration): boolean {
+ if (!program.isSourceFileDefaultLibrary(declaration.getSourceFile()) && declaration.heritageClauses) {
+ for (const heritageClause of declaration.heritageClauses) {
+ for (const type of heritageClause.types) {
+ const symbol = findSymbolFromHeritageType(checker, type);
+ if (symbol) {
+ const decl = symbol.valueDeclaration || (symbol.declarations && symbol.declarations[0]);
+ if (decl && program.isSourceFileDefaultLibrary(decl.getSourceFile())) {
+ return true;
+ }
+ }
+ }
+ }
+ }
+ return false;
+}
+
+function findSymbolFromHeritageType(checker: ts.TypeChecker, type: ts.ExpressionWithTypeArguments | ts.Expression | ts.PrivateIdentifier): ts.Symbol | null {
+ if (ts.isExpressionWithTypeArguments(type)) {
+ return findSymbolFromHeritageType(checker, type.expression);
+ }
+ if (ts.isIdentifier(type)) {
+ return getRealNodeSymbol(checker, type)[0];
+ }
+ if (ts.isPropertyAccessExpression(type)) {
+ return findSymbolFromHeritageType(checker, type.name);
+ }
+ return null;
+}
+
/**
* Returns the node's symbol and the `import` node (if the symbol resolved from a different module)
*/
@@ -708,7 +799,7 @@ function getRealNodeSymbol(checker: ts.TypeChecker, node: ts.Node): [ts.Symbol |
// (2) when the aliased symbol is originating from an import.
//
function shouldSkipAlias(node: ts.Node, declaration: ts.Node): boolean {
- if (node.kind !== ts.SyntaxKind.Identifier) {
+ if (!ts.isShorthandPropertyAssignment(node) && node.kind !== ts.SyntaxKind.Identifier) {
return false;
}
if (node.parent === declaration) {
@@ -733,7 +824,12 @@ function getRealNodeSymbol(checker: ts.TypeChecker, node: ts.Node): [ts.Symbol |
const { parent } = node;
- let symbol = checker.getSymbolAtLocation(node);
+ let symbol = (
+ ts.isShorthandPropertyAssignment(node)
+ ? checker.getShorthandAssignmentValueSymbol(node)
+ : checker.getSymbolAtLocation(node)
+ );
+
let importNode: ts.Declaration | null = null;
// If this is an alias, and the request came at the declaration location
// get the aliased symbol instead. This allows for goto def on an import e.g.
diff --git a/build/lib/tslint/abstractGlobalsRule.js b/build/lib/tslint/abstractGlobalsRule.js
deleted file mode 100644
index 1566c0aa57605..0000000000000
--- a/build/lib/tslint/abstractGlobalsRule.js
+++ /dev/null
@@ -1,45 +0,0 @@
-"use strict";
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-Object.defineProperty(exports, "__esModule", { value: true });
-const Lint = require("tslint");
-class AbstractGlobalsRuleWalker extends Lint.RuleWalker {
- constructor(file, program, opts, _config) {
- super(file, opts);
- this.program = program;
- this._config = _config;
- }
- visitIdentifier(node) {
- if (this.getDisallowedGlobals().some(disallowedGlobal => disallowedGlobal === node.text)) {
- if (this._config.allowed && this._config.allowed.some(allowed => allowed === node.text)) {
- return; // override
- }
- const checker = this.program.getTypeChecker();
- const symbol = checker.getSymbolAtLocation(node);
- if (symbol) {
- const declarations = symbol.declarations;
- if (Array.isArray(declarations) && symbol.declarations.some(declaration => {
- if (declaration) {
- const parent = declaration.parent;
- if (parent) {
- const sourceFile = parent.getSourceFile();
- if (sourceFile) {
- const fileName = sourceFile.fileName;
- if (fileName && fileName.indexOf(this.getDefinitionPattern()) >= 0) {
- return true;
- }
- }
- }
- }
- return false;
- })) {
- this.addFailureAtNode(node, `Cannot use global '${node.text}' in '${this._config.target}'`);
- }
- }
- }
- super.visitIdentifier(node);
- }
-}
-exports.AbstractGlobalsRuleWalker = AbstractGlobalsRuleWalker;
diff --git a/build/lib/tslint/abstractGlobalsRule.ts b/build/lib/tslint/abstractGlobalsRule.ts
deleted file mode 100644
index 543720455c3b7..0000000000000
--- a/build/lib/tslint/abstractGlobalsRule.ts
+++ /dev/null
@@ -1,57 +0,0 @@
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-
-import * as ts from 'typescript';
-import * as Lint from 'tslint';
-
-interface AbstractGlobalsRuleConfig {
- target: string;
- allowed: string[];
-}
-
-export abstract class AbstractGlobalsRuleWalker extends Lint.RuleWalker {
-
- constructor(file: ts.SourceFile, private program: ts.Program, opts: Lint.IOptions, private _config: AbstractGlobalsRuleConfig) {
- super(file, opts);
- }
-
- protected abstract getDisallowedGlobals(): string[];
-
- protected abstract getDefinitionPattern(): string;
-
- visitIdentifier(node: ts.Identifier) {
- if (this.getDisallowedGlobals().some(disallowedGlobal => disallowedGlobal === node.text)) {
- if (this._config.allowed && this._config.allowed.some(allowed => allowed === node.text)) {
- return; // override
- }
-
- const checker = this.program.getTypeChecker();
- const symbol = checker.getSymbolAtLocation(node);
- if (symbol) {
- const declarations = symbol.declarations;
- if (Array.isArray(declarations) && symbol.declarations.some(declaration => {
- if (declaration) {
- const parent = declaration.parent;
- if (parent) {
- const sourceFile = parent.getSourceFile();
- if (sourceFile) {
- const fileName = sourceFile.fileName;
- if (fileName && fileName.indexOf(this.getDefinitionPattern()) >= 0) {
- return true;
- }
- }
- }
- }
-
- return false;
- })) {
- this.addFailureAtNode(node, `Cannot use global '${node.text}' in '${this._config.target}'`);
- }
- }
- }
-
- super.visitIdentifier(node);
- }
-}
diff --git a/build/lib/tslint/duplicateImportsRule.js b/build/lib/tslint/duplicateImportsRule.js
deleted file mode 100644
index f336095fc6a20..0000000000000
--- a/build/lib/tslint/duplicateImportsRule.js
+++ /dev/null
@@ -1,32 +0,0 @@
-"use strict";
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-Object.defineProperty(exports, "__esModule", { value: true });
-const path_1 = require("path");
-const Lint = require("tslint");
-class Rule extends Lint.Rules.AbstractRule {
- apply(sourceFile) {
- return this.applyWithWalker(new ImportPatterns(sourceFile, this.getOptions()));
- }
-}
-exports.Rule = Rule;
-class ImportPatterns extends Lint.RuleWalker {
- constructor(file, opts) {
- super(file, opts);
- this.imports = Object.create(null);
- }
- visitImportDeclaration(node) {
- let path = node.moduleSpecifier.getText();
- // remove quotes
- path = path.slice(1, -1);
- if (path[0] === '.') {
- path = path_1.join(path_1.dirname(node.getSourceFile().fileName), path);
- }
- if (this.imports[path]) {
- this.addFailure(this.createFailure(node.getStart(), node.getWidth(), `Duplicate imports for '${path}'.`));
- }
- this.imports[path] = true;
- }
-}
diff --git a/build/lib/tslint/duplicateImportsRule.ts b/build/lib/tslint/duplicateImportsRule.ts
deleted file mode 100644
index c648084be1db0..0000000000000
--- a/build/lib/tslint/duplicateImportsRule.ts
+++ /dev/null
@@ -1,40 +0,0 @@
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-
-import * as ts from 'typescript';
-import { join, dirname } from 'path';
-import * as Lint from 'tslint';
-
-export class Rule extends Lint.Rules.AbstractRule {
- public apply(sourceFile: ts.SourceFile): Lint.RuleFailure[] {
- return this.applyWithWalker(new ImportPatterns(sourceFile, this.getOptions()));
- }
-}
-
-class ImportPatterns extends Lint.RuleWalker {
-
- private imports: { [path: string]: boolean; } = Object.create(null);
-
- constructor(file: ts.SourceFile, opts: Lint.IOptions) {
- super(file, opts);
- }
-
- protected visitImportDeclaration(node: ts.ImportDeclaration): void {
- let path = node.moduleSpecifier.getText();
-
- // remove quotes
- path = path.slice(1, -1);
-
- if (path[0] === '.') {
- path = join(dirname(node.getSourceFile().fileName), path);
- }
-
- if (this.imports[path]) {
- this.addFailure(this.createFailure(node.getStart(), node.getWidth(), `Duplicate imports for '${path}'.`));
- }
-
- this.imports[path] = true;
- }
-}
diff --git a/build/lib/tslint/importPatternsRule.js b/build/lib/tslint/importPatternsRule.js
deleted file mode 100644
index a64f5a6967877..0000000000000
--- a/build/lib/tslint/importPatternsRule.js
+++ /dev/null
@@ -1,70 +0,0 @@
-"use strict";
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-Object.defineProperty(exports, "__esModule", { value: true });
-const ts = require("typescript");
-const Lint = require("tslint");
-const minimatch = require("minimatch");
-const path_1 = require("path");
-class Rule extends Lint.Rules.AbstractRule {
- apply(sourceFile) {
- const configs = this.getOptions().ruleArguments;
- for (const config of configs) {
- if (minimatch(sourceFile.fileName, config.target)) {
- return this.applyWithWalker(new ImportPatterns(sourceFile, this.getOptions(), config));
- }
- }
- return [];
- }
-}
-exports.Rule = Rule;
-class ImportPatterns extends Lint.RuleWalker {
- constructor(file, opts, _config) {
- super(file, opts);
- this._config = _config;
- }
- visitImportEqualsDeclaration(node) {
- if (node.moduleReference.kind === ts.SyntaxKind.ExternalModuleReference) {
- this._validateImport(node.moduleReference.expression.getText(), node);
- }
- }
- visitImportDeclaration(node) {
- this._validateImport(node.moduleSpecifier.getText(), node);
- }
- visitCallExpression(node) {
- super.visitCallExpression(node);
- // import('foo') statements inside the code
- if (node.expression.kind === ts.SyntaxKind.ImportKeyword) {
- const [path] = node.arguments;
- this._validateImport(path.getText(), node);
- }
- }
- _validateImport(path, node) {
- // remove quotes
- path = path.slice(1, -1);
- // resolve relative paths
- if (path[0] === '.') {
- path = path_1.join(this.getSourceFile().fileName, path);
- }
- let restrictions;
- if (typeof this._config.restrictions === 'string') {
- restrictions = [this._config.restrictions];
- }
- else {
- restrictions = this._config.restrictions;
- }
- let matched = false;
- for (const pattern of restrictions) {
- if (minimatch(path, pattern)) {
- matched = true;
- break;
- }
- }
- if (!matched) {
- // None of the restrictions matched
- this.addFailure(this.createFailure(node.getStart(), node.getWidth(), `Imports violates '${restrictions.join(' or ')}' restrictions. See https://github.com/Microsoft/vscode/wiki/Code-Organization`));
- }
- }
-}
diff --git a/build/lib/tslint/importPatternsRule.ts b/build/lib/tslint/importPatternsRule.ts
deleted file mode 100644
index 6e545a6be1605..0000000000000
--- a/build/lib/tslint/importPatternsRule.ts
+++ /dev/null
@@ -1,87 +0,0 @@
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-
-import * as ts from 'typescript';
-import * as Lint from 'tslint';
-import * as minimatch from 'minimatch';
-import { join } from 'path';
-
-interface ImportPatternsConfig {
- target: string;
- restrictions: string | string[];
-}
-
-export class Rule extends Lint.Rules.AbstractRule {
- public apply(sourceFile: ts.SourceFile): Lint.RuleFailure[] {
-
- const configs = this.getOptions().ruleArguments;
-
-
- for (const config of configs) {
- if (minimatch(sourceFile.fileName, config.target)) {
- return this.applyWithWalker(new ImportPatterns(sourceFile, this.getOptions(), config));
- }
- }
-
- return [];
- }
-}
-
-class ImportPatterns extends Lint.RuleWalker {
-
- constructor(file: ts.SourceFile, opts: Lint.IOptions, private _config: ImportPatternsConfig) {
- super(file, opts);
- }
-
- protected visitImportEqualsDeclaration(node: ts.ImportEqualsDeclaration): void {
- if (node.moduleReference.kind === ts.SyntaxKind.ExternalModuleReference) {
- this._validateImport(node.moduleReference.expression.getText(), node);
- }
- }
-
- protected visitImportDeclaration(node: ts.ImportDeclaration): void {
- this._validateImport(node.moduleSpecifier.getText(), node);
- }
-
- protected visitCallExpression(node: ts.CallExpression): void {
- super.visitCallExpression(node);
-
- // import('foo') statements inside the code
- if (node.expression.kind === ts.SyntaxKind.ImportKeyword) {
- const [path] = node.arguments;
- this._validateImport(path.getText(), node);
- }
- }
-
- private _validateImport(path: string, node: ts.Node): void {
- // remove quotes
- path = path.slice(1, -1);
-
- // resolve relative paths
- if (path[0] === '.') {
- path = join(this.getSourceFile().fileName, path);
- }
-
- let restrictions: string[];
- if (typeof this._config.restrictions === 'string') {
- restrictions = [this._config.restrictions];
- } else {
- restrictions = this._config.restrictions;
- }
-
- let matched = false;
- for (const pattern of restrictions) {
- if (minimatch(path, pattern)) {
- matched = true;
- break;
- }
- }
-
- if (!matched) {
- // None of the restrictions matched
- this.addFailure(this.createFailure(node.getStart(), node.getWidth(), `Imports violates '${restrictions.join(' or ')}' restrictions. See https://github.com/Microsoft/vscode/wiki/Code-Organization`));
- }
- }
-}
diff --git a/build/lib/tslint/layeringRule.js b/build/lib/tslint/layeringRule.js
deleted file mode 100644
index 21a7c9a16d947..0000000000000
--- a/build/lib/tslint/layeringRule.js
+++ /dev/null
@@ -1,83 +0,0 @@
-"use strict";
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-Object.defineProperty(exports, "__esModule", { value: true });
-const ts = require("typescript");
-const Lint = require("tslint");
-const path_1 = require("path");
-class Rule extends Lint.Rules.AbstractRule {
- apply(sourceFile) {
- const parts = path_1.dirname(sourceFile.fileName).split(/\\|\//);
- const ruleArgs = this.getOptions().ruleArguments[0];
- let config;
- for (let i = parts.length - 1; i >= 0; i--) {
- if (ruleArgs[parts[i]]) {
- config = {
- allowed: new Set(ruleArgs[parts[i]]).add(parts[i]),
- disallowed: new Set()
- };
- Object.keys(ruleArgs).forEach(key => {
- if (!config.allowed.has(key)) {
- config.disallowed.add(key);
- }
- });
- break;
- }
- }
- if (!config) {
- return [];
- }
- return this.applyWithWalker(new LayeringRule(sourceFile, config, this.getOptions()));
- }
-}
-exports.Rule = Rule;
-class LayeringRule extends Lint.RuleWalker {
- constructor(file, config, opts) {
- super(file, opts);
- this._config = config;
- }
- visitImportEqualsDeclaration(node) {
- if (node.moduleReference.kind === ts.SyntaxKind.ExternalModuleReference) {
- this._validateImport(node.moduleReference.expression.getText(), node);
- }
- }
- visitImportDeclaration(node) {
- this._validateImport(node.moduleSpecifier.getText(), node);
- }
- visitCallExpression(node) {
- super.visitCallExpression(node);
- // import('foo') statements inside the code
- if (node.expression.kind === ts.SyntaxKind.ImportKeyword) {
- const [path] = node.arguments;
- this._validateImport(path.getText(), node);
- }
- }
- _validateImport(path, node) {
- // remove quotes
- path = path.slice(1, -1);
- if (path[0] === '.') {
- path = path_1.join(path_1.dirname(node.getSourceFile().fileName), path);
- }
- const parts = path_1.dirname(path).split(/\\|\//);
- for (let i = parts.length - 1; i >= 0; i--) {
- const part = parts[i];
- if (this._config.allowed.has(part)) {
- // GOOD - same layer
- return;
- }
- if (this._config.disallowed.has(part)) {
- // BAD - wrong layer
- const message = `Bad layering. You are not allowed to access '${part}' from here, allowed layers are: [${LayeringRule._print(this._config.allowed)}]`;
- this.addFailure(this.createFailure(node.getStart(), node.getWidth(), message));
- return;
- }
- }
- }
- static _print(set) {
- const r = [];
- set.forEach(e => r.push(e));
- return r.join(', ');
- }
-}
diff --git a/build/lib/tslint/layeringRule.ts b/build/lib/tslint/layeringRule.ts
deleted file mode 100644
index dc0053fe8de17..0000000000000
--- a/build/lib/tslint/layeringRule.ts
+++ /dev/null
@@ -1,105 +0,0 @@
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-
-import * as ts from 'typescript';
-import * as Lint from 'tslint';
-import { join, dirname } from 'path';
-
-interface Config {
- allowed: Set;
- disallowed: Set;
-}
-
-export class Rule extends Lint.Rules.AbstractRule {
- public apply(sourceFile: ts.SourceFile): Lint.RuleFailure[] {
-
- const parts = dirname(sourceFile.fileName).split(/\\|\//);
- const ruleArgs = this.getOptions().ruleArguments[0];
-
- let config: Config | undefined;
- for (let i = parts.length - 1; i >= 0; i--) {
- if (ruleArgs[parts[i]]) {
- config = {
- allowed: new Set(ruleArgs[parts[i]]).add(parts[i]),
- disallowed: new Set()
- };
- Object.keys(ruleArgs).forEach(key => {
- if (!config!.allowed.has(key)) {
- config!.disallowed.add(key);
- }
- });
- break;
- }
- }
-
- if (!config) {
- return [];
- }
-
- return this.applyWithWalker(new LayeringRule(sourceFile, config, this.getOptions()));
- }
-}
-
-class LayeringRule extends Lint.RuleWalker {
-
- private _config: Config;
-
- constructor(file: ts.SourceFile, config: Config, opts: Lint.IOptions) {
- super(file, opts);
- this._config = config;
- }
-
- protected visitImportEqualsDeclaration(node: ts.ImportEqualsDeclaration): void {
- if (node.moduleReference.kind === ts.SyntaxKind.ExternalModuleReference) {
- this._validateImport(node.moduleReference.expression.getText(), node);
- }
- }
-
- protected visitImportDeclaration(node: ts.ImportDeclaration): void {
- this._validateImport(node.moduleSpecifier.getText(), node);
- }
-
- protected visitCallExpression(node: ts.CallExpression): void {
- super.visitCallExpression(node);
-
- // import('foo') statements inside the code
- if (node.expression.kind === ts.SyntaxKind.ImportKeyword) {
- const [path] = node.arguments;
- this._validateImport(path.getText(), node);
- }
- }
-
- private _validateImport(path: string, node: ts.Node): void {
- // remove quotes
- path = path.slice(1, -1);
-
- if (path[0] === '.') {
- path = join(dirname(node.getSourceFile().fileName), path);
- }
-
- const parts = dirname(path).split(/\\|\//);
- for (let i = parts.length - 1; i >= 0; i--) {
- const part = parts[i];
-
- if (this._config.allowed.has(part)) {
- // GOOD - same layer
- return;
- }
-
- if (this._config.disallowed.has(part)) {
- // BAD - wrong layer
- const message = `Bad layering. You are not allowed to access '${part}' from here, allowed layers are: [${LayeringRule._print(this._config.allowed)}]`;
- this.addFailure(this.createFailure(node.getStart(), node.getWidth(), message));
- return;
- }
- }
- }
-
- static _print(set: Set): string {
- const r: string[] = [];
- set.forEach(e => r.push(e));
- return r.join(', ');
- }
-}
diff --git a/build/lib/tslint/noDomGlobalsRule.js b/build/lib/tslint/noDomGlobalsRule.js
deleted file mode 100644
index a83ac8f7f5958..0000000000000
--- a/build/lib/tslint/noDomGlobalsRule.js
+++ /dev/null
@@ -1,34 +0,0 @@
-"use strict";
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-Object.defineProperty(exports, "__esModule", { value: true });
-const Lint = require("tslint");
-const minimatch = require("minimatch");
-const abstractGlobalsRule_1 = require("./abstractGlobalsRule");
-class Rule extends Lint.Rules.TypedRule {
- applyWithProgram(sourceFile, program) {
- const configs = this.getOptions().ruleArguments;
- for (const config of configs) {
- if (minimatch(sourceFile.fileName, config.target)) {
- return this.applyWithWalker(new NoDOMGlobalsRuleWalker(sourceFile, program, this.getOptions(), config));
- }
- }
- return [];
- }
-}
-exports.Rule = Rule;
-class NoDOMGlobalsRuleWalker extends abstractGlobalsRule_1.AbstractGlobalsRuleWalker {
- getDefinitionPattern() {
- return 'lib.dom.d.ts';
- }
- getDisallowedGlobals() {
- // intentionally not complete
- return [
- "window",
- "document",
- "HTMLElement"
- ];
- }
-}
diff --git a/build/lib/tslint/noDomGlobalsRule.ts b/build/lib/tslint/noDomGlobalsRule.ts
deleted file mode 100644
index df9e67bf78b6f..0000000000000
--- a/build/lib/tslint/noDomGlobalsRule.ts
+++ /dev/null
@@ -1,45 +0,0 @@
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-
-import * as ts from 'typescript';
-import * as Lint from 'tslint';
-import * as minimatch from 'minimatch';
-import { AbstractGlobalsRuleWalker } from './abstractGlobalsRule';
-
-interface NoDOMGlobalsRuleConfig {
- target: string;
- allowed: string[];
-}
-
-export class Rule extends Lint.Rules.TypedRule {
-
- applyWithProgram(sourceFile: ts.SourceFile, program: ts.Program): Lint.RuleFailure[] {
- const configs = this.getOptions().ruleArguments;
-
- for (const config of configs) {
- if (minimatch(sourceFile.fileName, config.target)) {
- return this.applyWithWalker(new NoDOMGlobalsRuleWalker(sourceFile, program, this.getOptions(), config));
- }
- }
-
- return [];
- }
-}
-
-class NoDOMGlobalsRuleWalker extends AbstractGlobalsRuleWalker {
-
- getDefinitionPattern(): string {
- return 'lib.dom.d.ts';
- }
-
- getDisallowedGlobals(): string[] {
- // intentionally not complete
- return [
- "window",
- "document",
- "HTMLElement"
- ];
- }
-}
diff --git a/build/lib/tslint/noNewBufferRule.js b/build/lib/tslint/noNewBufferRule.js
deleted file mode 100644
index ae9b02d457ec4..0000000000000
--- a/build/lib/tslint/noNewBufferRule.js
+++ /dev/null
@@ -1,22 +0,0 @@
-"use strict";
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-Object.defineProperty(exports, "__esModule", { value: true });
-const ts = require("typescript");
-const Lint = require("tslint");
-class Rule extends Lint.Rules.AbstractRule {
- apply(sourceFile) {
- return this.applyWithWalker(new NewBufferRuleWalker(sourceFile, this.getOptions()));
- }
-}
-exports.Rule = Rule;
-class NewBufferRuleWalker extends Lint.RuleWalker {
- visitNewExpression(node) {
- if (node.expression.kind === ts.SyntaxKind.Identifier && node.expression && node.expression.text === 'Buffer') {
- this.addFailureAtNode(node, '`new Buffer` is deprecated. Consider Buffer.From or Buffer.alloc instead.');
- }
- super.visitNewExpression(node);
- }
-}
diff --git a/build/lib/tslint/noNewBufferRule.ts b/build/lib/tslint/noNewBufferRule.ts
deleted file mode 100644
index 7b0dd43e5383a..0000000000000
--- a/build/lib/tslint/noNewBufferRule.ts
+++ /dev/null
@@ -1,23 +0,0 @@
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-
-import * as ts from 'typescript';
-import * as Lint from 'tslint';
-
-export class Rule extends Lint.Rules.AbstractRule {
- apply(sourceFile: ts.SourceFile): Lint.RuleFailure[] {
- return this.applyWithWalker(new NewBufferRuleWalker(sourceFile, this.getOptions()));
- }
-}
-
-class NewBufferRuleWalker extends Lint.RuleWalker {
- visitNewExpression(node: ts.NewExpression) {
- if (node.expression.kind === ts.SyntaxKind.Identifier && node.expression && (node.expression as ts.Identifier).text === 'Buffer') {
- this.addFailureAtNode(node, '`new Buffer` is deprecated. Consider Buffer.From or Buffer.alloc instead.');
- }
-
- super.visitNewExpression(node);
- }
-}
\ No newline at end of file
diff --git a/build/lib/tslint/noNlsInStandaloneEditorRule.js b/build/lib/tslint/noNlsInStandaloneEditorRule.js
deleted file mode 100644
index affd4cc837034..0000000000000
--- a/build/lib/tslint/noNlsInStandaloneEditorRule.js
+++ /dev/null
@@ -1,54 +0,0 @@
-"use strict";
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-Object.defineProperty(exports, "__esModule", { value: true });
-const ts = require("typescript");
-const Lint = require("tslint");
-const path_1 = require("path");
-class Rule extends Lint.Rules.AbstractRule {
- apply(sourceFile) {
- if (/vs(\/|\\)editor(\/|\\)standalone(\/|\\)/.test(sourceFile.fileName)
- || /vs(\/|\\)editor(\/|\\)common(\/|\\)standalone(\/|\\)/.test(sourceFile.fileName)
- || /vs(\/|\\)editor(\/|\\)editor.api/.test(sourceFile.fileName)
- || /vs(\/|\\)editor(\/|\\)editor.main/.test(sourceFile.fileName)
- || /vs(\/|\\)editor(\/|\\)editor.worker/.test(sourceFile.fileName)) {
- return this.applyWithWalker(new NoNlsInStandaloneEditorRuleWalker(sourceFile, this.getOptions()));
- }
- return [];
- }
-}
-exports.Rule = Rule;
-class NoNlsInStandaloneEditorRuleWalker extends Lint.RuleWalker {
- constructor(file, opts) {
- super(file, opts);
- }
- visitImportEqualsDeclaration(node) {
- if (node.moduleReference.kind === ts.SyntaxKind.ExternalModuleReference) {
- this._validateImport(node.moduleReference.expression.getText(), node);
- }
- }
- visitImportDeclaration(node) {
- this._validateImport(node.moduleSpecifier.getText(), node);
- }
- visitCallExpression(node) {
- super.visitCallExpression(node);
- // import('foo') statements inside the code
- if (node.expression.kind === ts.SyntaxKind.ImportKeyword) {
- const [path] = node.arguments;
- this._validateImport(path.getText(), node);
- }
- }
- _validateImport(path, node) {
- // remove quotes
- path = path.slice(1, -1);
- // resolve relative paths
- if (path[0] === '.') {
- path = path_1.join(this.getSourceFile().fileName, path);
- }
- if (/vs(\/|\\)nls/.test(path)) {
- this.addFailure(this.createFailure(node.getStart(), node.getWidth(), `Not allowed to import vs/nls in standalone editor modules. Use standaloneStrings.ts`));
- }
- }
-}
diff --git a/build/lib/tslint/noNlsInStandaloneEditorRule.ts b/build/lib/tslint/noNlsInStandaloneEditorRule.ts
deleted file mode 100644
index ae23d74d784d5..0000000000000
--- a/build/lib/tslint/noNlsInStandaloneEditorRule.ts
+++ /dev/null
@@ -1,67 +0,0 @@
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-
-import * as ts from 'typescript';
-import * as Lint from 'tslint';
-import { join } from 'path';
-
-export class Rule extends Lint.Rules.AbstractRule {
- public apply(sourceFile: ts.SourceFile): Lint.RuleFailure[] {
- if (
- /vs(\/|\\)editor(\/|\\)standalone(\/|\\)/.test(sourceFile.fileName)
- || /vs(\/|\\)editor(\/|\\)common(\/|\\)standalone(\/|\\)/.test(sourceFile.fileName)
- || /vs(\/|\\)editor(\/|\\)editor.api/.test(sourceFile.fileName)
- || /vs(\/|\\)editor(\/|\\)editor.main/.test(sourceFile.fileName)
- || /vs(\/|\\)editor(\/|\\)editor.worker/.test(sourceFile.fileName)
- ) {
- return this.applyWithWalker(new NoNlsInStandaloneEditorRuleWalker(sourceFile, this.getOptions()));
- }
-
- return [];
- }
-}
-
-class NoNlsInStandaloneEditorRuleWalker extends Lint.RuleWalker {
-
- constructor(file: ts.SourceFile, opts: Lint.IOptions) {
- super(file, opts);
- }
-
- protected visitImportEqualsDeclaration(node: ts.ImportEqualsDeclaration): void {
- if (node.moduleReference.kind === ts.SyntaxKind.ExternalModuleReference) {
- this._validateImport(node.moduleReference.expression.getText(), node);
- }
- }
-
- protected visitImportDeclaration(node: ts.ImportDeclaration): void {
- this._validateImport(node.moduleSpecifier.getText(), node);
- }
-
- protected visitCallExpression(node: ts.CallExpression): void {
- super.visitCallExpression(node);
-
- // import('foo') statements inside the code
- if (node.expression.kind === ts.SyntaxKind.ImportKeyword) {
- const [path] = node.arguments;
- this._validateImport(path.getText(), node);
- }
- }
-
- private _validateImport(path: string, node: ts.Node): void {
- // remove quotes
- path = path.slice(1, -1);
-
- // resolve relative paths
- if (path[0] === '.') {
- path = join(this.getSourceFile().fileName, path);
- }
-
- if (
- /vs(\/|\\)nls/.test(path)
- ) {
- this.addFailure(this.createFailure(node.getStart(), node.getWidth(), `Not allowed to import vs/nls in standalone editor modules. Use standaloneStrings.ts`));
- }
- }
-}
diff --git a/build/lib/tslint/noNodejsGlobalsRule.js b/build/lib/tslint/noNodejsGlobalsRule.js
deleted file mode 100644
index 8c36fa342c273..0000000000000
--- a/build/lib/tslint/noNodejsGlobalsRule.js
+++ /dev/null
@@ -1,41 +0,0 @@
-"use strict";
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-Object.defineProperty(exports, "__esModule", { value: true });
-const Lint = require("tslint");
-const minimatch = require("minimatch");
-const abstractGlobalsRule_1 = require("./abstractGlobalsRule");
-class Rule extends Lint.Rules.TypedRule {
- applyWithProgram(sourceFile, program) {
- const configs = this.getOptions().ruleArguments;
- for (const config of configs) {
- if (minimatch(sourceFile.fileName, config.target)) {
- return this.applyWithWalker(new NoNodejsGlobalsRuleWalker(sourceFile, program, this.getOptions(), config));
- }
- }
- return [];
- }
-}
-exports.Rule = Rule;
-class NoNodejsGlobalsRuleWalker extends abstractGlobalsRule_1.AbstractGlobalsRuleWalker {
- getDefinitionPattern() {
- return '@types/node';
- }
- getDisallowedGlobals() {
- // https://nodejs.org/api/globals.html#globals_global_objects
- return [
- "NodeJS",
- "Buffer",
- "__dirname",
- "__filename",
- "clearImmediate",
- "exports",
- "global",
- "module",
- "process",
- "setImmediate"
- ];
- }
-}
diff --git a/build/lib/tslint/noNodejsGlobalsRule.ts b/build/lib/tslint/noNodejsGlobalsRule.ts
deleted file mode 100644
index 7e5767d857086..0000000000000
--- a/build/lib/tslint/noNodejsGlobalsRule.ts
+++ /dev/null
@@ -1,52 +0,0 @@
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-
-import * as ts from 'typescript';
-import * as Lint from 'tslint';
-import * as minimatch from 'minimatch';
-import { AbstractGlobalsRuleWalker } from './abstractGlobalsRule';
-
-interface NoNodejsGlobalsConfig {
- target: string;
- allowed: string[];
-}
-
-export class Rule extends Lint.Rules.TypedRule {
-
- applyWithProgram(sourceFile: ts.SourceFile, program: ts.Program): Lint.RuleFailure[] {
- const configs = this.getOptions().ruleArguments;
-
- for (const config of configs) {
- if (minimatch(sourceFile.fileName, config.target)) {
- return this.applyWithWalker(new NoNodejsGlobalsRuleWalker(sourceFile, program, this.getOptions(), config));
- }
- }
-
- return [];
- }
-}
-
-class NoNodejsGlobalsRuleWalker extends AbstractGlobalsRuleWalker {
-
- getDefinitionPattern(): string {
- return '@types/node';
- }
-
- getDisallowedGlobals(): string[] {
- // https://nodejs.org/api/globals.html#globals_global_objects
- return [
- "NodeJS",
- "Buffer",
- "__dirname",
- "__filename",
- "clearImmediate",
- "exports",
- "global",
- "module",
- "process",
- "setImmediate"
- ];
- }
-}
diff --git a/build/lib/tslint/noStandaloneEditorRule.js b/build/lib/tslint/noStandaloneEditorRule.js
deleted file mode 100644
index 44b70a6a8d7bb..0000000000000
--- a/build/lib/tslint/noStandaloneEditorRule.js
+++ /dev/null
@@ -1,55 +0,0 @@
-"use strict";
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-Object.defineProperty(exports, "__esModule", { value: true });
-const ts = require("typescript");
-const Lint = require("tslint");
-const path_1 = require("path");
-class Rule extends Lint.Rules.AbstractRule {
- apply(sourceFile) {
- if (/vs(\/|\\)editor/.test(sourceFile.fileName)) {
- // the vs/editor folder is allowed to use the standalone editor
- return [];
- }
- return this.applyWithWalker(new NoStandaloneEditorRuleWalker(sourceFile, this.getOptions()));
- }
-}
-exports.Rule = Rule;
-class NoStandaloneEditorRuleWalker extends Lint.RuleWalker {
- constructor(file, opts) {
- super(file, opts);
- }
- visitImportEqualsDeclaration(node) {
- if (node.moduleReference.kind === ts.SyntaxKind.ExternalModuleReference) {
- this._validateImport(node.moduleReference.expression.getText(), node);
- }
- }
- visitImportDeclaration(node) {
- this._validateImport(node.moduleSpecifier.getText(), node);
- }
- visitCallExpression(node) {
- super.visitCallExpression(node);
- // import('foo') statements inside the code
- if (node.expression.kind === ts.SyntaxKind.ImportKeyword) {
- const [path] = node.arguments;
- this._validateImport(path.getText(), node);
- }
- }
- _validateImport(path, node) {
- // remove quotes
- path = path.slice(1, -1);
- // resolve relative paths
- if (path[0] === '.') {
- path = path_1.join(this.getSourceFile().fileName, path);
- }
- if (/vs(\/|\\)editor(\/|\\)standalone(\/|\\)/.test(path)
- || /vs(\/|\\)editor(\/|\\)common(\/|\\)standalone(\/|\\)/.test(path)
- || /vs(\/|\\)editor(\/|\\)editor.api/.test(path)
- || /vs(\/|\\)editor(\/|\\)editor.main/.test(path)
- || /vs(\/|\\)editor(\/|\\)editor.worker/.test(path)) {
- this.addFailure(this.createFailure(node.getStart(), node.getWidth(), `Not allowed to import standalone editor modules. See https://github.com/Microsoft/vscode/wiki/Code-Organization`));
- }
- }
-}
diff --git a/build/lib/tslint/noStandaloneEditorRule.ts b/build/lib/tslint/noStandaloneEditorRule.ts
deleted file mode 100644
index 713b94097d4c0..0000000000000
--- a/build/lib/tslint/noStandaloneEditorRule.ts
+++ /dev/null
@@ -1,65 +0,0 @@
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-
-import * as ts from 'typescript';
-import * as Lint from 'tslint';
-import { join } from 'path';
-
-export class Rule extends Lint.Rules.AbstractRule {
- public apply(sourceFile: ts.SourceFile): Lint.RuleFailure[] {
- if (/vs(\/|\\)editor/.test(sourceFile.fileName)) {
- // the vs/editor folder is allowed to use the standalone editor
- return [];
- }
- return this.applyWithWalker(new NoStandaloneEditorRuleWalker(sourceFile, this.getOptions()));
- }
-}
-
-class NoStandaloneEditorRuleWalker extends Lint.RuleWalker {
-
- constructor(file: ts.SourceFile, opts: Lint.IOptions) {
- super(file, opts);
- }
-
- protected visitImportEqualsDeclaration(node: ts.ImportEqualsDeclaration): void {
- if (node.moduleReference.kind === ts.SyntaxKind.ExternalModuleReference) {
- this._validateImport(node.moduleReference.expression.getText(), node);
- }
- }
-
- protected visitImportDeclaration(node: ts.ImportDeclaration): void {
- this._validateImport(node.moduleSpecifier.getText(), node);
- }
-
- protected visitCallExpression(node: ts.CallExpression): void {
- super.visitCallExpression(node);
-
- // import('foo') statements inside the code
- if (node.expression.kind === ts.SyntaxKind.ImportKeyword) {
- const [path] = node.arguments;
- this._validateImport(path.getText(), node);
- }
- }
-
- private _validateImport(path: string, node: ts.Node): void {
- // remove quotes
- path = path.slice(1, -1);
-
- // resolve relative paths
- if (path[0] === '.') {
- path = join(this.getSourceFile().fileName, path);
- }
-
- if (
- /vs(\/|\\)editor(\/|\\)standalone(\/|\\)/.test(path)
- || /vs(\/|\\)editor(\/|\\)common(\/|\\)standalone(\/|\\)/.test(path)
- || /vs(\/|\\)editor(\/|\\)editor.api/.test(path)
- || /vs(\/|\\)editor(\/|\\)editor.main/.test(path)
- || /vs(\/|\\)editor(\/|\\)editor.worker/.test(path)
- ) {
- this.addFailure(this.createFailure(node.getStart(), node.getWidth(), `Not allowed to import standalone editor modules. See https://github.com/Microsoft/vscode/wiki/Code-Organization`));
- }
- }
-}
diff --git a/build/lib/tslint/noUnexternalizedStringsRule.js b/build/lib/tslint/noUnexternalizedStringsRule.js
deleted file mode 100644
index a1183ce68e767..0000000000000
--- a/build/lib/tslint/noUnexternalizedStringsRule.js
+++ /dev/null
@@ -1,183 +0,0 @@
-"use strict";
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-Object.defineProperty(exports, "__esModule", { value: true });
-const ts = require("typescript");
-const Lint = require("tslint");
-/**
- * Implementation of the no-unexternalized-strings rule.
- */
-class Rule extends Lint.Rules.AbstractRule {
- apply(sourceFile) {
- if (/\.d.ts$/.test(sourceFile.fileName)) {
- return [];
- }
- return this.applyWithWalker(new NoUnexternalizedStringsRuleWalker(sourceFile, this.getOptions()));
- }
-}
-exports.Rule = Rule;
-function isStringLiteral(node) {
- return node && node.kind === ts.SyntaxKind.StringLiteral;
-}
-function isObjectLiteral(node) {
- return node && node.kind === ts.SyntaxKind.ObjectLiteralExpression;
-}
-function isPropertyAssignment(node) {
- return node && node.kind === ts.SyntaxKind.PropertyAssignment;
-}
-class NoUnexternalizedStringsRuleWalker extends Lint.RuleWalker {
- constructor(file, opts) {
- super(file, opts);
- this.signatures = Object.create(null);
- this.ignores = Object.create(null);
- this.messageIndex = undefined;
- this.keyIndex = undefined;
- this.usedKeys = Object.create(null);
- const options = this.getOptions();
- const first = options && options.length > 0 ? options[0] : null;
- if (first) {
- if (Array.isArray(first.signatures)) {
- first.signatures.forEach((signature) => this.signatures[signature] = true);
- }
- if (Array.isArray(first.ignores)) {
- first.ignores.forEach((ignore) => this.ignores[ignore] = true);
- }
- if (typeof first.messageIndex !== 'undefined') {
- this.messageIndex = first.messageIndex;
- }
- if (typeof first.keyIndex !== 'undefined') {
- this.keyIndex = first.keyIndex;
- }
- }
- }
- visitSourceFile(node) {
- super.visitSourceFile(node);
- Object.keys(this.usedKeys).forEach(key => {
- // Keys are quoted.
- let identifier = key.substr(1, key.length - 2);
- if (!NoUnexternalizedStringsRuleWalker.IDENTIFIER.test(identifier)) {
- let occurrence = this.usedKeys[key][0];
- this.addFailure(this.createFailure(occurrence.key.getStart(), occurrence.key.getWidth(), `The key ${occurrence.key.getText()} doesn't conform to a valid localize identifier`));
- }
- const occurrences = this.usedKeys[key];
- if (occurrences.length > 1) {
- occurrences.forEach(occurrence => {
- this.addFailure((this.createFailure(occurrence.key.getStart(), occurrence.key.getWidth(), `Duplicate key ${occurrence.key.getText()} with different message value.`)));
- });
- }
- });
- }
- visitStringLiteral(node) {
- this.checkStringLiteral(node);
- super.visitStringLiteral(node);
- }
- checkStringLiteral(node) {
- const text = node.getText();
- const doubleQuoted = text.length >= 2 && text[0] === NoUnexternalizedStringsRuleWalker.DOUBLE_QUOTE && text[text.length - 1] === NoUnexternalizedStringsRuleWalker.DOUBLE_QUOTE;
- const info = this.findDescribingParent(node);
- // Ignore strings in import and export nodes.
- if (info && info.isImport && doubleQuoted) {
- const fix = [
- Lint.Replacement.replaceFromTo(node.getStart(), 1, '\''),
- Lint.Replacement.replaceFromTo(node.getStart() + text.length - 1, 1, '\''),
- ];
- this.addFailureAtNode(node, NoUnexternalizedStringsRuleWalker.ImportFailureMessage, fix);
- return;
- }
- const callInfo = info ? info.callInfo : null;
- const functionName = callInfo ? callInfo.callExpression.expression.getText() : null;
- if (functionName && this.ignores[functionName]) {
- return;
- }
- if (doubleQuoted && (!callInfo || callInfo.argIndex === -1 || !this.signatures[functionName])) {
- const s = node.getText();
- const fix = [
- Lint.Replacement.replaceFromTo(node.getStart(), node.getWidth(), `nls.localize('KEY-${s.substring(1, s.length - 1)}', ${s})`),
- ];
- this.addFailure(this.createFailure(node.getStart(), node.getWidth(), `Unexternalized string found: ${node.getText()}`, fix));
- return;
- }
- // We have a single quoted string outside a localize function name.
- if (!doubleQuoted && !this.signatures[functionName]) {
- return;
- }
- // We have a string that is a direct argument into the localize call.
- const keyArg = callInfo && callInfo.argIndex === this.keyIndex
- ? callInfo.callExpression.arguments[this.keyIndex]
- : null;
- if (keyArg) {
- if (isStringLiteral(keyArg)) {
- this.recordKey(keyArg, this.messageIndex && callInfo ? callInfo.callExpression.arguments[this.messageIndex] : undefined);
- }
- else if (isObjectLiteral(keyArg)) {
- for (const property of keyArg.properties) {
- if (isPropertyAssignment(property)) {
- const name = property.name.getText();
- if (name === 'key') {
- const initializer = property.initializer;
- if (isStringLiteral(initializer)) {
- this.recordKey(initializer, this.messageIndex && callInfo ? callInfo.callExpression.arguments[this.messageIndex] : undefined);
- }
- break;
- }
- }
- }
- }
- }
- const messageArg = callInfo.callExpression.arguments[this.messageIndex];
- if (messageArg && messageArg.kind !== ts.SyntaxKind.StringLiteral) {
- this.addFailure(this.createFailure(messageArg.getStart(), messageArg.getWidth(), `Message argument to '${callInfo.callExpression.expression.getText()}' must be a string literal.`));
- return;
- }
- }
- recordKey(keyNode, messageNode) {
- const text = keyNode.getText();
- // We have an empty key
- if (text.match(/(['"]) *\1/)) {
- if (messageNode) {
- this.addFailureAtNode(keyNode, `Key is empty for message: ${messageNode.getText()}`);
- }
- else {
- this.addFailureAtNode(keyNode, `Key is empty.`);
- }
- return;
- }
- let occurrences = this.usedKeys[text];
- if (!occurrences) {
- occurrences = [];
- this.usedKeys[text] = occurrences;
- }
- if (messageNode) {
- if (occurrences.some(pair => pair.message ? pair.message.getText() === messageNode.getText() : false)) {
- return;
- }
- }
- occurrences.push({ key: keyNode, message: messageNode });
- }
- findDescribingParent(node) {
- let parent;
- while ((parent = node.parent)) {
- const kind = parent.kind;
- if (kind === ts.SyntaxKind.CallExpression) {
- const callExpression = parent;
- return { callInfo: { callExpression: callExpression, argIndex: callExpression.arguments.indexOf(node) } };
- }
- else if (kind === ts.SyntaxKind.ImportEqualsDeclaration || kind === ts.SyntaxKind.ImportDeclaration || kind === ts.SyntaxKind.ExportDeclaration) {
- return { isImport: true };
- }
- else if (kind === ts.SyntaxKind.VariableDeclaration || kind === ts.SyntaxKind.FunctionDeclaration || kind === ts.SyntaxKind.PropertyDeclaration
- || kind === ts.SyntaxKind.MethodDeclaration || kind === ts.SyntaxKind.VariableDeclarationList || kind === ts.SyntaxKind.InterfaceDeclaration
- || kind === ts.SyntaxKind.ClassDeclaration || kind === ts.SyntaxKind.EnumDeclaration || kind === ts.SyntaxKind.ModuleDeclaration
- || kind === ts.SyntaxKind.TypeAliasDeclaration || kind === ts.SyntaxKind.SourceFile) {
- return null;
- }
- node = parent;
- }
- return null;
- }
-}
-NoUnexternalizedStringsRuleWalker.ImportFailureMessage = 'Do not use double quotes for imports.';
-NoUnexternalizedStringsRuleWalker.DOUBLE_QUOTE = '"';
-NoUnexternalizedStringsRuleWalker.IDENTIFIER = /^[_a-zA-Z0-9][ .\-_a-zA-Z0-9]*$/;
diff --git a/build/lib/tslint/noUnexternalizedStringsRule.ts b/build/lib/tslint/noUnexternalizedStringsRule.ts
deleted file mode 100644
index d896e4fabe2ee..0000000000000
--- a/build/lib/tslint/noUnexternalizedStringsRule.ts
+++ /dev/null
@@ -1,222 +0,0 @@
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-
-import * as ts from 'typescript';
-import * as Lint from 'tslint';
-
-/**
- * Implementation of the no-unexternalized-strings rule.
- */
-export class Rule extends Lint.Rules.AbstractRule {
- public apply(sourceFile: ts.SourceFile): Lint.RuleFailure[] {
- if (/\.d.ts$/.test(sourceFile.fileName)) {
- return [];
- }
- return this.applyWithWalker(new NoUnexternalizedStringsRuleWalker(sourceFile, this.getOptions()));
- }
-}
-
-interface Map {
- [key: string]: V;
-}
-
-interface UnexternalizedStringsOptions {
- signatures?: string[];
- messageIndex?: number;
- keyIndex?: number;
- ignores?: string[];
-}
-
-function isStringLiteral(node: ts.Node): node is ts.StringLiteral {
- return node && node.kind === ts.SyntaxKind.StringLiteral;
-}
-
-function isObjectLiteral(node: ts.Node): node is ts.ObjectLiteralExpression {
- return node && node.kind === ts.SyntaxKind.ObjectLiteralExpression;
-}
-
-function isPropertyAssignment(node: ts.Node): node is ts.PropertyAssignment {
- return node && node.kind === ts.SyntaxKind.PropertyAssignment;
-}
-
-interface KeyMessagePair {
- key: ts.StringLiteral;
- message: ts.Node | undefined;
-}
-
-class NoUnexternalizedStringsRuleWalker extends Lint.RuleWalker {
-
- private static ImportFailureMessage = 'Do not use double quotes for imports.';
-
- private static DOUBLE_QUOTE: string = '"';
-
- private signatures: Map;
- private messageIndex: number | undefined;
- private keyIndex: number | undefined;
- private ignores: Map;
-
- private usedKeys: Map;
-
- constructor(file: ts.SourceFile, opts: Lint.IOptions) {
- super(file, opts);
- this.signatures = Object.create(null);
- this.ignores = Object.create(null);
- this.messageIndex = undefined;
- this.keyIndex = undefined;
- this.usedKeys = Object.create(null);
- const options: any[] = this.getOptions();
- const first: UnexternalizedStringsOptions = options && options.length > 0 ? options[0] : null;
- if (first) {
- if (Array.isArray(first.signatures)) {
- first.signatures.forEach((signature: string) => this.signatures[signature] = true);
- }
- if (Array.isArray(first.ignores)) {
- first.ignores.forEach((ignore: string) => this.ignores[ignore] = true);
- }
- if (typeof first.messageIndex !== 'undefined') {
- this.messageIndex = first.messageIndex;
- }
- if (typeof first.keyIndex !== 'undefined') {
- this.keyIndex = first.keyIndex;
- }
- }
- }
-
- private static IDENTIFIER = /^[_a-zA-Z0-9][ .\-_a-zA-Z0-9]*$/;
- protected visitSourceFile(node: ts.SourceFile): void {
- super.visitSourceFile(node);
- Object.keys(this.usedKeys).forEach(key => {
- // Keys are quoted.
- let identifier = key.substr(1, key.length - 2);
- if (!NoUnexternalizedStringsRuleWalker.IDENTIFIER.test(identifier)) {
- let occurrence = this.usedKeys[key][0];
- this.addFailure(this.createFailure(occurrence.key.getStart(), occurrence.key.getWidth(), `The key ${occurrence.key.getText()} doesn't conform to a valid localize identifier`));
- }
- const occurrences = this.usedKeys[key];
- if (occurrences.length > 1) {
- occurrences.forEach(occurrence => {
- this.addFailure((this.createFailure(occurrence.key.getStart(), occurrence.key.getWidth(), `Duplicate key ${occurrence.key.getText()} with different message value.`)));
- });
- }
- });
- }
-
- protected visitStringLiteral(node: ts.StringLiteral): void {
- this.checkStringLiteral(node);
- super.visitStringLiteral(node);
- }
-
- private checkStringLiteral(node: ts.StringLiteral): void {
- const text = node.getText();
- const doubleQuoted = text.length >= 2 && text[0] === NoUnexternalizedStringsRuleWalker.DOUBLE_QUOTE && text[text.length - 1] === NoUnexternalizedStringsRuleWalker.DOUBLE_QUOTE;
- const info = this.findDescribingParent(node);
- // Ignore strings in import and export nodes.
- if (info && info.isImport && doubleQuoted) {
- const fix = [
- Lint.Replacement.replaceFromTo(node.getStart(), 1, '\''),
- Lint.Replacement.replaceFromTo(node.getStart() + text.length - 1, 1, '\''),
- ];
- this.addFailureAtNode(
- node,
- NoUnexternalizedStringsRuleWalker.ImportFailureMessage,
- fix
- );
- return;
- }
- const callInfo = info ? info.callInfo : null;
- const functionName = callInfo ? callInfo.callExpression.expression.getText() : null;
- if (functionName && this.ignores[functionName]) {
- return;
- }
-
- if (doubleQuoted && (!callInfo || callInfo.argIndex === -1 || !this.signatures[functionName!])) {
- const s = node.getText();
- const fix = [
- Lint.Replacement.replaceFromTo(node.getStart(), node.getWidth(), `nls.localize('KEY-${s.substring(1, s.length - 1)}', ${s})`),
- ];
- this.addFailure(this.createFailure(node.getStart(), node.getWidth(), `Unexternalized string found: ${node.getText()}`, fix));
- return;
- }
- // We have a single quoted string outside a localize function name.
- if (!doubleQuoted && !this.signatures[functionName!]) {
- return;
- }
- // We have a string that is a direct argument into the localize call.
- const keyArg: ts.Expression | null = callInfo && callInfo.argIndex === this.keyIndex
- ? callInfo.callExpression.arguments[this.keyIndex]
- : null;
- if (keyArg) {
- if (isStringLiteral(keyArg)) {
- this.recordKey(keyArg, this.messageIndex && callInfo ? callInfo.callExpression.arguments[this.messageIndex] : undefined);
- } else if (isObjectLiteral(keyArg)) {
- for (const property of keyArg.properties) {
- if (isPropertyAssignment(property)) {
- const name = property.name.getText();
- if (name === 'key') {
- const initializer = property.initializer;
- if (isStringLiteral(initializer)) {
- this.recordKey(initializer, this.messageIndex && callInfo ? callInfo.callExpression.arguments[this.messageIndex] : undefined);
- }
- break;
- }
- }
- }
- }
- }
-
- const messageArg = callInfo!.callExpression.arguments[this.messageIndex!];
-
- if (messageArg && messageArg.kind !== ts.SyntaxKind.StringLiteral) {
- this.addFailure(this.createFailure(
- messageArg.getStart(), messageArg.getWidth(),
- `Message argument to '${callInfo!.callExpression.expression.getText()}' must be a string literal.`));
- return;
- }
- }
-
- private recordKey(keyNode: ts.StringLiteral, messageNode: ts.Node | undefined) {
- const text = keyNode.getText();
- // We have an empty key
- if (text.match(/(['"]) *\1/)) {
- if (messageNode) {
- this.addFailureAtNode(keyNode, `Key is empty for message: ${messageNode.getText()}`);
- } else {
- this.addFailureAtNode(keyNode, `Key is empty.`);
- }
- return;
- }
- let occurrences: KeyMessagePair[] = this.usedKeys[text];
- if (!occurrences) {
- occurrences = [];
- this.usedKeys[text] = occurrences;
- }
- if (messageNode) {
- if (occurrences.some(pair => pair.message ? pair.message.getText() === messageNode.getText() : false)) {
- return;
- }
- }
- occurrences.push({ key: keyNode, message: messageNode });
- }
-
- private findDescribingParent(node: ts.Node): { callInfo?: { callExpression: ts.CallExpression, argIndex: number }, isImport?: boolean; } | null {
- let parent: ts.Node;
- while ((parent = node.parent)) {
- const kind = parent.kind;
- if (kind === ts.SyntaxKind.CallExpression) {
- const callExpression = parent as ts.CallExpression;
- return { callInfo: { callExpression: callExpression, argIndex: callExpression.arguments.indexOf(node) } };
- } else if (kind === ts.SyntaxKind.ImportEqualsDeclaration || kind === ts.SyntaxKind.ImportDeclaration || kind === ts.SyntaxKind.ExportDeclaration) {
- return { isImport: true };
- } else if (kind === ts.SyntaxKind.VariableDeclaration || kind === ts.SyntaxKind.FunctionDeclaration || kind === ts.SyntaxKind.PropertyDeclaration
- || kind === ts.SyntaxKind.MethodDeclaration || kind === ts.SyntaxKind.VariableDeclarationList || kind === ts.SyntaxKind.InterfaceDeclaration
- || kind === ts.SyntaxKind.ClassDeclaration || kind === ts.SyntaxKind.EnumDeclaration || kind === ts.SyntaxKind.ModuleDeclaration
- || kind === ts.SyntaxKind.TypeAliasDeclaration || kind === ts.SyntaxKind.SourceFile) {
- return null;
- }
- node = parent;
- }
- return null;
- }
-}
diff --git a/build/lib/tslint/translationRemindRule.js b/build/lib/tslint/translationRemindRule.js
deleted file mode 100644
index 2234094421bb9..0000000000000
--- a/build/lib/tslint/translationRemindRule.js
+++ /dev/null
@@ -1,62 +0,0 @@
-"use strict";
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-Object.defineProperty(exports, "__esModule", { value: true });
-const Lint = require("tslint");
-const fs = require("fs");
-class Rule extends Lint.Rules.AbstractRule {
- apply(sourceFile) {
- return this.applyWithWalker(new TranslationRemindRuleWalker(sourceFile, this.getOptions()));
- }
-}
-exports.Rule = Rule;
-class TranslationRemindRuleWalker extends Lint.RuleWalker {
- constructor(file, opts) {
- super(file, opts);
- }
- visitImportDeclaration(node) {
- const declaration = node.moduleSpecifier.getText();
- if (declaration !== `'${TranslationRemindRuleWalker.NLS_MODULE}'`) {
- return;
- }
- this.visitImportLikeDeclaration(node);
- }
- visitImportEqualsDeclaration(node) {
- const reference = node.moduleReference.getText();
- if (reference !== `require('${TranslationRemindRuleWalker.NLS_MODULE}')`) {
- return;
- }
- this.visitImportLikeDeclaration(node);
- }
- visitImportLikeDeclaration(node) {
- const currentFile = node.getSourceFile().fileName;
- const matchService = currentFile.match(/vs\/workbench\/services\/\w+/);
- const matchPart = currentFile.match(/vs\/workbench\/contrib\/\w+/);
- if (!matchService && !matchPart) {
- return;
- }
- const resource = matchService ? matchService[0] : matchPart[0];
- let resourceDefined = false;
- let json;
- try {
- json = fs.readFileSync('./build/lib/i18n.resources.json', 'utf8');
- }
- catch (e) {
- console.error('[translation-remind rule]: File with resources to pull from Transifex was not found. Aborting translation resource check for newly defined workbench part/service.');
- return;
- }
- const workbenchResources = JSON.parse(json).workbench;
- workbenchResources.forEach((existingResource) => {
- if (existingResource.name === resource) {
- resourceDefined = true;
- return;
- }
- });
- if (!resourceDefined) {
- this.addFailureAtNode(node, `Please add '${resource}' to ./build/lib/i18n.resources.json file to use translations here.`);
- }
- }
-}
-TranslationRemindRuleWalker.NLS_MODULE = 'vs/nls';
diff --git a/build/lib/tslint/translationRemindRule.ts b/build/lib/tslint/translationRemindRule.ts
deleted file mode 100644
index e2671599d3c0a..0000000000000
--- a/build/lib/tslint/translationRemindRule.ts
+++ /dev/null
@@ -1,73 +0,0 @@
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-
-import * as ts from 'typescript';
-import * as Lint from 'tslint';
-import * as fs from 'fs';
-
-export class Rule extends Lint.Rules.AbstractRule {
- public apply(sourceFile: ts.SourceFile): Lint.RuleFailure[] {
- return this.applyWithWalker(new TranslationRemindRuleWalker(sourceFile, this.getOptions()));
- }
-}
-
-class TranslationRemindRuleWalker extends Lint.RuleWalker {
-
- private static NLS_MODULE: string = 'vs/nls';
-
- constructor(file: ts.SourceFile, opts: Lint.IOptions) {
- super(file, opts);
- }
-
- protected visitImportDeclaration(node: ts.ImportDeclaration): void {
- const declaration = node.moduleSpecifier.getText();
- if (declaration !== `'${TranslationRemindRuleWalker.NLS_MODULE}'`) {
- return;
- }
-
- this.visitImportLikeDeclaration(node);
- }
-
- protected visitImportEqualsDeclaration(node: ts.ImportEqualsDeclaration): void {
- const reference = node.moduleReference.getText();
- if (reference !== `require('${TranslationRemindRuleWalker.NLS_MODULE}')`) {
- return;
- }
-
- this.visitImportLikeDeclaration(node);
- }
-
- private visitImportLikeDeclaration(node: ts.ImportDeclaration | ts.ImportEqualsDeclaration) {
- const currentFile = node.getSourceFile().fileName;
- const matchService = currentFile.match(/vs\/workbench\/services\/\w+/);
- const matchPart = currentFile.match(/vs\/workbench\/contrib\/\w+/);
- if (!matchService && !matchPart) {
- return;
- }
-
- const resource = matchService ? matchService[0] : matchPart![0];
- let resourceDefined = false;
-
- let json;
- try {
- json = fs.readFileSync('./build/lib/i18n.resources.json', 'utf8');
- } catch (e) {
- console.error('[translation-remind rule]: File with resources to pull from Transifex was not found. Aborting translation resource check for newly defined workbench part/service.');
- return;
- }
- const workbenchResources = JSON.parse(json).workbench;
-
- workbenchResources.forEach((existingResource: any) => {
- if (existingResource.name === resource) {
- resourceDefined = true;
- return;
- }
- });
-
- if (!resourceDefined) {
- this.addFailureAtNode(node, `Please add '${resource}' to ./build/lib/i18n.resources.json file to use translations here.`);
- }
- }
-}
diff --git a/build/lib/util.js b/build/lib/util.js
index 1888bfed2e448..e552a036f89bd 100644
--- a/build/lib/util.js
+++ b/build/lib/util.js
@@ -4,6 +4,7 @@
*--------------------------------------------------------------------------------------------*/
'use strict';
Object.defineProperty(exports, "__esModule", { value: true });
+exports.getElectronVersion = exports.streamToPromise = exports.versionStringToNumber = exports.filter = exports.rebase = exports.getVersion = exports.ensureDir = exports.rreddir = exports.rimraf = exports.stripSourceMappingURL = exports.loadSourcemaps = exports.cleanNodeModules = exports.skipDirectories = exports.toFileUri = exports.setExecutableBit = exports.fixWin32DirectoryPermissions = exports.incremental = void 0;
const es = require("event-stream");
const debounce = require("debounce");
const _filter = require("gulp-filter");
@@ -13,6 +14,7 @@ const fs = require("fs");
const _rimraf = require("rimraf");
const git = require("./git");
const VinylFile = require("vinyl");
+const root = path.dirname(path.dirname(__dirname));
const NoCancellationToken = { isCancellationRequested: () => false };
function incremental(streamProvider, initial, supportsCancellation) {
const input = es.through();
@@ -136,7 +138,7 @@ function loadSourcemaps() {
version: '3',
names: [],
mappings: '',
- sources: [f.relative.replace(/\//g, '/')],
+ sources: [f.relative],
sourcesContent: [contents]
};
cb(undefined, f);
@@ -185,6 +187,31 @@ function rimraf(dir) {
return result;
}
exports.rimraf = rimraf;
+function _rreaddir(dirPath, prepend, result) {
+ const entries = fs.readdirSync(dirPath, { withFileTypes: true });
+ for (const entry of entries) {
+ if (entry.isDirectory()) {
+ _rreaddir(path.join(dirPath, entry.name), `${prepend}/${entry.name}`, result);
+ }
+ else {
+ result.push(`${prepend}/${entry.name}`);
+ }
+ }
+}
+function rreddir(dirPath) {
+ let result = [];
+ _rreaddir(dirPath, '', result);
+ return result;
+}
+exports.rreddir = rreddir;
+function ensureDir(dirPath) {
+ if (fs.existsSync(dirPath)) {
+ return;
+ }
+ ensureDir(path.dirname(dirPath));
+ fs.mkdirSync(dirPath);
+}
+exports.ensureDir = ensureDir;
function getVersion(root) {
let version = process.env['BUILD_SOURCEVERSION'];
if (!version || !/^[0-9a-f]{40}$/i.test(version)) {
@@ -229,3 +256,9 @@ function streamToPromise(stream) {
});
}
exports.streamToPromise = streamToPromise;
+function getElectronVersion() {
+ const yarnrc = fs.readFileSync(path.join(root, '.yarnrc'), 'utf8');
+ const target = /^target "(.*)"$/m.exec(yarnrc)[1];
+ return target;
+}
+exports.getElectronVersion = getElectronVersion;
diff --git a/build/lib/util.ts b/build/lib/util.ts
index ae598d5f2cfd0..035c7e95ea300 100644
--- a/build/lib/util.ts
+++ b/build/lib/util.ts
@@ -18,6 +18,8 @@ import * as VinylFile from 'vinyl';
import { ThroughStream } from 'through';
import * as sm from 'source-map';
+const root = path.dirname(path.dirname(__dirname));
+
export interface ICancellationToken {
isCancellationRequested(): boolean;
}
@@ -184,7 +186,7 @@ export function loadSourcemaps(): NodeJS.ReadWriteStream {
version: '3',
names: [],
mappings: '',
- sources: [f.relative.replace(/\//g, '/')],
+ sources: [f.relative],
sourcesContent: [contents]
};
@@ -243,6 +245,31 @@ export function rimraf(dir: string): () => Promise {
return result;
}
+function _rreaddir(dirPath: string, prepend: string, result: string[]): void {
+ const entries = fs.readdirSync(dirPath, { withFileTypes: true });
+ for (const entry of entries) {
+ if (entry.isDirectory()) {
+ _rreaddir(path.join(dirPath, entry.name), `${prepend}/${entry.name}`, result);
+ } else {
+ result.push(`${prepend}/${entry.name}`);
+ }
+ }
+}
+
+export function rreddir(dirPath: string): string[] {
+ let result: string[] = [];
+ _rreaddir(dirPath, '', result);
+ return result;
+}
+
+export function ensureDir(dirPath: string): void {
+ if (fs.existsSync(dirPath)) {
+ return;
+ }
+ ensureDir(path.dirname(dirPath));
+ fs.mkdirSync(dirPath);
+}
+
export function getVersion(root: string): string | undefined {
let version = process.env['BUILD_SOURCEVERSION'];
@@ -293,3 +320,9 @@ export function streamToPromise(stream: NodeJS.ReadWriteStream): Promise {
stream.on('end', () => c());
});
}
+
+export function getElectronVersion(): string {
+ const yarnrc = fs.readFileSync(path.join(root, '.yarnrc'), 'utf8');
+ const target = /^target "(.*)"$/m.exec(yarnrc)![1];
+ return target;
+}
diff --git a/build/lib/watch/watch-win32.js b/build/lib/watch/watch-win32.js
index d0cd307ba1690..91c0eae75659f 100644
--- a/build/lib/watch/watch-win32.js
+++ b/build/lib/watch/watch-win32.js
@@ -25,7 +25,6 @@ function watch(root) {
var child = cp.spawn(watcherPath, [root]);
child.stdout.on('data', function (data) {
- // @ts-ignore
var lines = data.toString('utf8').split('\n');
for (var i = 0; i < lines.length; i++) {
var line = lines[i].trim();
@@ -47,7 +46,6 @@ function watch(root) {
path: changePathFull,
base: root
});
- //@ts-ignore
file.event = toChangeType(changeType);
result.emit('data', file);
}
@@ -106,4 +104,4 @@ module.exports = function (pattern, options) {
});
}))
.pipe(rebase);
-};
\ No newline at end of file
+};
diff --git a/build/lib/watch/yarn.lock b/build/lib/watch/yarn.lock
index 3f330da1764b4..edbfe1f3121d8 100644
--- a/build/lib/watch/yarn.lock
+++ b/build/lib/watch/yarn.lock
@@ -230,9 +230,9 @@ for-own@^0.1.4:
for-in "^1.0.1"
fsevents@~2.1.1:
- version "2.1.1"
- resolved "https://registry.yarnpkg.com/fsevents/-/fsevents-2.1.1.tgz#74c64e21df71721845d0c44fe54b7f56b82995a9"
- integrity sha512-4FRPXWETxtigtJW/gxzEDsX1LVbPAM93VleB83kZB+ellqbHMkyt2aJfuzNLRvFPnGi6bcE5SvfxgbXPeKteJw==
+ version "2.1.2"
+ resolved "https://registry.yarnpkg.com/fsevents/-/fsevents-2.1.2.tgz#4c0a1fb34bc68e543b4b82a9ec392bfbda840805"
+ integrity sha512-R4wDiBwZ0KzpgOWetKDug1FZcYhqYnUYKtfZYt4mD5SBz76q0KR4Q9o7GIPamsVPGmW3EYPPJ0dOOjvx32ldZA==
glob-base@^0.3.0:
version "0.3.0"
@@ -258,9 +258,9 @@ glob-parent@^3.0.1:
path-dirname "^1.0.0"
glob-parent@~5.1.0:
- version "5.1.0"
- resolved "https://registry.yarnpkg.com/glob-parent/-/glob-parent-5.1.0.tgz#5f4c1d1e748d30cd73ad2944b3577a81b081e8c2"
- integrity sha512-qjtRgnIVmOfnKUE3NJAQEdk+lKrxfw8t5ke7SXtfMTHcjsBfOfWXCQfdb30zfDoZQ2IRSIiidmjtbHZPZ++Ihw==
+ version "5.1.1"
+ resolved "https://registry.yarnpkg.com/glob-parent/-/glob-parent-5.1.1.tgz#b6c1ef417c4e5663ea498f1c45afac6916bbc229"
+ integrity sha512-FnI+VGOpnlGHWZxthPGR+QhR78fuiK0sNLkHQv+bL9fQi57lNNdquIbna/WrfROrolq8GK5Ek6BiMwqL/voRYQ==
dependencies:
is-glob "^4.0.1"
@@ -405,9 +405,9 @@ kind-of@^3.0.2:
is-buffer "^1.1.5"
kind-of@^6.0.0:
- version "6.0.2"
- resolved "https://registry.yarnpkg.com/kind-of/-/kind-of-6.0.2.tgz#01146b36a6218e64e58f3a8d66de5d7fc6f6d051"
- integrity sha512-s5kLOcnH0XqDO+FvuaLX8DDjZ18CGFk7VygH40QoKPUQhW4e2rvM0rwUq0t8IQDOwYSeLK01U90OjzBTme2QqA==
+ version "6.0.3"
+ resolved "https://registry.yarnpkg.com/kind-of/-/kind-of-6.0.3.tgz#07c05034a6c349fa06e24fa35aa76db4580ce4dd"
+ integrity sha512-dcS1ul+9tmeD95T+x28/ehLgd9mENa3LsvDTtzm3vyBEO7RPptvAD+t44WVXaUjTBRcrpFeFlC8WCruUR456hw==
math-random@^1.0.1:
version "1.0.4"
@@ -479,9 +479,9 @@ path-is-absolute@^1.0.1:
integrity sha1-F0uSaHNVNP+8es5r9TpanhtcX18=
picomatch@^2.0.4:
- version "2.1.0"
- resolved "https://registry.yarnpkg.com/picomatch/-/picomatch-2.1.0.tgz#0fd042f568d08b1ad9ff2d3ec0f0bfb3cb80e177"
- integrity sha512-uhnEDzAbrcJ8R3g2fANnSuXZMBtkpSjxTTgn2LeSiQlfmq72enQJWdQllXW24MBLYnA1SBD2vfvx2o0Zw3Ielw==
+ version "2.2.2"
+ resolved "https://registry.yarnpkg.com/picomatch/-/picomatch-2.2.2.tgz#21f333e9b6b8eaff02468f5146ea406d345f4dad"
+ integrity sha512-q0M/9eZHzmr0AulXyPwNfZjtwZ/RBZlbN3K3CErVrk50T2ASYI7Bye0EvekFY3IP1Nt2DHu0re+V2ZHIpMkuWg==
pify@^2.3.0:
version "2.3.0"
@@ -530,9 +530,9 @@ randomatic@^3.0.0:
math-random "^1.0.1"
readable-stream@^2.0.2, readable-stream@^2.2.2, readable-stream@^2.3.5:
- version "2.3.6"
- resolved "https://registry.yarnpkg.com/readable-stream/-/readable-stream-2.3.6.tgz#b11c27d88b8ff1fbe070643cf94b0c79ae1b0aaf"
- integrity sha512-tQtKA9WIAhBF3+VLAseyMqZeBjW0AHJoxOtYqSUZNJxauErmLbVm2FW1y+J/YA9dUrAC39ITejlZWhVIwawkKw==
+ version "2.3.7"
+ resolved "https://registry.yarnpkg.com/readable-stream/-/readable-stream-2.3.7.tgz#1eca1cf711aef814c04f62252a36a62f6cb23b57"
+ integrity sha512-Ebho8K4jIbHAxnuxi7o42OrZgF/ZTNcsZj6nRKyUmkhLFq8CHItp/fy6hQZuZmP/n3yZ9VBUbp4zz/mX8hmYPw==
dependencies:
core-util-is "~1.0.0"
inherits "~2.0.3"
diff --git a/build/monaco/ThirdPartyNotices.txt b/build/monaco/ThirdPartyNotices.txt
index 1de70ddaab6c7..8b488daf191a9 100644
--- a/build/monaco/ThirdPartyNotices.txt
+++ b/build/monaco/ThirdPartyNotices.txt
@@ -33,32 +33,6 @@ USE OR OTHER DEALINGS IN THE SOFTWARE.
END OF nodejs path library NOTICES AND INFORMATION
-%% promise-polyfill version 8.1.0 (https://github.com/taylorhakes/promise-polyfill)
-=========================================
-Copyright (c) 2014 Taylor Hakes
-Copyright (c) 2014 Forbes Lindesay
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in
-all copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
-THE SOFTWARE.
-=========================================
-END OF winjs NOTICES AND INFORMATION
-
-
%% string_scorer version 0.1.20 (https://github.com/joshaven/string_score)
diff --git a/build/monaco/api.js b/build/monaco/api.js
index a429cd66cde20..1de24d4065c8a 100644
--- a/build/monaco/api.js
+++ b/build/monaco/api.js
@@ -4,12 +4,13 @@
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
Object.defineProperty(exports, "__esModule", { value: true });
+exports.execute = exports.run3 = exports.DeclarationResolver = exports.FSProvider = exports.RECIPE_PATH = void 0;
const fs = require("fs");
const ts = require("typescript");
const path = require("path");
const fancyLog = require("fancy-log");
const ansiColors = require("ansi-colors");
-const dtsv = '2';
+const dtsv = '3';
const tsfmt = require('../../tsfmt.json');
const SRC = path.join(__dirname, '../../src');
exports.RECIPE_PATH = path.join(__dirname, './monaco.d.ts.recipe');
@@ -135,11 +136,12 @@ function getMassagedTopLevelDeclarationText(sourceFile, declaration, importName,
}
else {
const memberName = member.name.text;
+ const memberAccess = (memberName.indexOf('.') >= 0 ? `['${memberName}']` : `.${memberName}`);
if (isStatic(member)) {
- usage.push(`a = ${staticTypeName}.${memberName};`);
+ usage.push(`a = ${staticTypeName}${memberAccess};`);
}
else {
- usage.push(`a = (<${instanceTypeName}>b).${memberName};`);
+ usage.push(`a = (<${instanceTypeName}>b)${memberAccess};`);
}
}
}
@@ -148,12 +150,44 @@ function getMassagedTopLevelDeclarationText(sourceFile, declaration, importName,
}
});
}
+ else if (declaration.kind === ts.SyntaxKind.VariableStatement) {
+ const jsDoc = result.substr(0, declaration.getLeadingTriviaWidth(sourceFile));
+ if (jsDoc.indexOf('@monacodtsreplace') >= 0) {
+ const jsDocLines = jsDoc.split(/\r\n|\r|\n/);
+ let directives = [];
+ for (const jsDocLine of jsDocLines) {
+ const m = jsDocLine.match(/^\s*\* \/([^/]+)\/([^/]+)\/$/);
+ if (m) {
+ directives.push([new RegExp(m[1], 'g'), m[2]]);
+ }
+ }
+ // remove the jsdoc
+ result = result.substr(jsDoc.length);
+ if (directives.length > 0) {
+ // apply replace directives
+ const replacer = createReplacerFromDirectives(directives);
+ result = replacer(result);
+ }
+ }
+ }
result = result.replace(/export default /g, 'export ');
result = result.replace(/export declare /g, 'export ');
result = result.replace(/declare /g, '');
+ let lines = result.split(/\r\n|\r|\n/);
+ for (let i = 0; i < lines.length; i++) {
+ if (/\s*\*/.test(lines[i])) {
+ // very likely a comment
+ continue;
+ }
+ lines[i] = lines[i].replace(/"/g, '\'');
+ }
+ result = lines.join('\n');
if (declaration.kind === ts.SyntaxKind.EnumDeclaration) {
result = result.replace(/const enum/, 'enum');
- enums.push(result);
+ enums.push({
+ enumName: declaration.name.getText(sourceFile),
+ text: result
+ });
}
return result;
}
@@ -277,6 +311,14 @@ function format(text, endl) {
return result;
}
}
+function createReplacerFromDirectives(directives) {
+ return (str) => {
+ for (let i = 0; i < directives.length; i++) {
+ str = str.replace(directives[i][0], directives[i][1]);
+ }
+ return str;
+ };
+}
function createReplacer(data) {
data = data || '';
let rawDirectives = data.split(';');
@@ -292,12 +334,7 @@ function createReplacer(data) {
findStr = '\\b' + findStr + '\\b';
directives.push([new RegExp(findStr, 'g'), replaceStr]);
});
- return (str) => {
- for (let i = 0; i < directives.length; i++) {
- str = str.replace(directives[i][0], directives[i][1]);
- }
- return str;
- };
+ return createReplacerFromDirectives(directives);
}
function generateDeclarationFile(recipe, sourceFileGetter) {
const endl = /\r\n/.test(recipe) ? '\r\n' : '\n';
@@ -415,6 +452,15 @@ function generateDeclarationFile(recipe, sourceFileGetter) {
resultTxt = resultTxt.split(/\r\n|\n|\r/).join(endl);
resultTxt = format(resultTxt, endl);
resultTxt = resultTxt.split(/\r\n|\n|\r/).join(endl);
+ enums.sort((e1, e2) => {
+ if (e1.enumName < e2.enumName) {
+ return -1;
+ }
+ if (e1.enumName > e2.enumName) {
+ return 1;
+ }
+ return 0;
+ });
let resultEnums = [
'/*---------------------------------------------------------------------------------------------',
' * Copyright (c) Microsoft Corporation. All rights reserved.',
@@ -423,7 +469,7 @@ function generateDeclarationFile(recipe, sourceFileGetter) {
'',
'// THIS IS A GENERATED FILE. DO NOT EDIT DIRECTLY.',
''
- ].concat(enums).join(endl);
+ ].concat(enums.map(e => e.text)).join(endl);
resultEnums = resultEnums.split(/\r\n|\n|\r/).join(endl);
resultEnums = format(resultEnums, endl);
resultEnums = resultEnums.split(/\r\n|\n|\r/).join(endl);
diff --git a/build/monaco/api.ts b/build/monaco/api.ts
index ae058292344d7..f3542988a4b02 100644
--- a/build/monaco/api.ts
+++ b/build/monaco/api.ts
@@ -9,7 +9,7 @@ import * as path from 'path';
import * as fancyLog from 'fancy-log';
import * as ansiColors from 'ansi-colors';
-const dtsv = '2';
+const dtsv = '3';
const tsfmt = require('../../tsfmt.json');
@@ -138,7 +138,7 @@ function isDefaultExport(declaration: ts.InterfaceDeclaration | ts.ClassDeclarat
);
}
-function getMassagedTopLevelDeclarationText(sourceFile: ts.SourceFile, declaration: TSTopLevelDeclare, importName: string, usage: string[], enums: string[]): string {
+function getMassagedTopLevelDeclarationText(sourceFile: ts.SourceFile, declaration: TSTopLevelDeclare, importName: string, usage: string[], enums: IEnumEntry[]): string {
let result = getNodeText(sourceFile, declaration);
if (declaration.kind === ts.SyntaxKind.InterfaceDeclaration || declaration.kind === ts.SyntaxKind.ClassDeclaration) {
let interfaceDeclaration = declaration;
@@ -167,24 +167,56 @@ function getMassagedTopLevelDeclarationText(sourceFile: ts.SourceFile, declarati
result = result.replace(memberText, '');
} else {
const memberName = (member.name).text;
+ const memberAccess = (memberName.indexOf('.') >= 0 ? `['${memberName}']` : `.${memberName}`);
if (isStatic(member)) {
- usage.push(`a = ${staticTypeName}.${memberName};`);
+ usage.push(`a = ${staticTypeName}${memberAccess};`);
} else {
- usage.push(`a = (<${instanceTypeName}>b).${memberName};`);
+ usage.push(`a = (<${instanceTypeName}>b)${memberAccess};`);
}
}
} catch (err) {
// life..
}
});
+ } else if (declaration.kind === ts.SyntaxKind.VariableStatement) {
+ const jsDoc = result.substr(0, declaration.getLeadingTriviaWidth(sourceFile));
+ if (jsDoc.indexOf('@monacodtsreplace') >= 0) {
+ const jsDocLines = jsDoc.split(/\r\n|\r|\n/);
+ let directives: [RegExp, string][] = [];
+ for (const jsDocLine of jsDocLines) {
+ const m = jsDocLine.match(/^\s*\* \/([^/]+)\/([^/]+)\/$/);
+ if (m) {
+ directives.push([new RegExp(m[1], 'g'), m[2]]);
+ }
+ }
+ // remove the jsdoc
+ result = result.substr(jsDoc.length);
+ if (directives.length > 0) {
+ // apply replace directives
+ const replacer = createReplacerFromDirectives(directives);
+ result = replacer(result);
+ }
+ }
}
result = result.replace(/export default /g, 'export ');
result = result.replace(/export declare /g, 'export ');
result = result.replace(/declare /g, '');
+ let lines = result.split(/\r\n|\r|\n/);
+ for (let i = 0; i < lines.length; i++) {
+ if (/\s*\*/.test(lines[i])) {
+ // very likely a comment
+ continue;
+ }
+ lines[i] = lines[i].replace(/"/g, '\'');
+ }
+ result = lines.join('\n');
if (declaration.kind === ts.SyntaxKind.EnumDeclaration) {
result = result.replace(/const enum/, 'enum');
- enums.push(result);
+ enums.push({
+ enumName: declaration.name.getText(sourceFile),
+ text: result
+ });
}
return result;
@@ -324,6 +356,15 @@ function format(text: string, endl: string): string {
}
}
+function createReplacerFromDirectives(directives: [RegExp, string][]): (str: string) => string {
+ return (str: string) => {
+ for (let i = 0; i < directives.length; i++) {
+ str = str.replace(directives[i][0], directives[i][1]);
+ }
+ return str;
+ };
+}
+
function createReplacer(data: string): (str: string) => string {
data = data || '';
let rawDirectives = data.split(';');
@@ -341,12 +382,7 @@ function createReplacer(data: string): (str: string) => string {
directives.push([new RegExp(findStr, 'g'), replaceStr]);
});
- return (str: string) => {
- for (let i = 0; i < directives.length; i++) {
- str = str.replace(directives[i][0], directives[i][1]);
- }
- return str;
- };
+ return createReplacerFromDirectives(directives);
}
interface ITempResult {
@@ -355,6 +391,11 @@ interface ITempResult {
enums: string;
}
+interface IEnumEntry {
+ enumName: string;
+ text: string;
+}
+
function generateDeclarationFile(recipe: string, sourceFileGetter: SourceFileGetter): ITempResult | null {
const endl = /\r\n/.test(recipe) ? '\r\n' : '\n';
@@ -376,7 +417,7 @@ function generateDeclarationFile(recipe: string, sourceFileGetter: SourceFileGet
return importName;
};
- let enums: string[] = [];
+ let enums: IEnumEntry[] = [];
let version: string | null = null;
lines.forEach(line => {
@@ -492,6 +533,16 @@ function generateDeclarationFile(recipe: string, sourceFileGetter: SourceFileGet
resultTxt = format(resultTxt, endl);
resultTxt = resultTxt.split(/\r\n|\n|\r/).join(endl);
+ enums.sort((e1, e2) => {
+ if (e1.enumName < e2.enumName) {
+ return -1;
+ }
+ if (e1.enumName > e2.enumName) {
+ return 1;
+ }
+ return 0;
+ });
+
let resultEnums = [
'/*---------------------------------------------------------------------------------------------',
' * Copyright (c) Microsoft Corporation. All rights reserved.',
@@ -500,7 +551,7 @@ function generateDeclarationFile(recipe: string, sourceFileGetter: SourceFileGet
'',
'// THIS IS A GENERATED FILE. DO NOT EDIT DIRECTLY.',
''
- ].concat(enums).join(endl);
+ ].concat(enums.map(e => e.text)).join(endl);
resultEnums = resultEnums.split(/\r\n|\n|\r/).join(endl);
resultEnums = format(resultEnums, endl);
resultEnums = resultEnums.split(/\r\n|\n|\r/).join(endl);
diff --git a/build/monaco/esm.core.js b/build/monaco/esm.core.js
new file mode 100644
index 0000000000000..b84b5fb4f4122
--- /dev/null
+++ b/build/monaco/esm.core.js
@@ -0,0 +1,21 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+// Entry file for webpack bunlding.
+
+import * as monaco from 'monaco-editor-core';
+
+self.MonacoEnvironment = {
+ getWorkerUrl: function (moduleId, label) {
+ return './editor.worker.bundle.js';
+ }
+};
+
+monaco.editor.create(document.getElementById('container'), {
+ value: [
+ 'var hello = "hello world";'
+ ].join('\n'),
+ language: 'javascript'
+});
diff --git a/build/monaco/monaco.d.ts.recipe b/build/monaco/monaco.d.ts.recipe
index 900b1bcce6064..7288af94c9d99 100644
--- a/build/monaco/monaco.d.ts.recipe
+++ b/build/monaco/monaco.d.ts.recipe
@@ -3,12 +3,18 @@
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
-declare namespace monaco {
+declare let MonacoEnvironment: monaco.Environment | undefined;
- // THIS IS A GENERATED FILE. DO NOT EDIT DIRECTLY.
+declare namespace monaco {
export type Thenable = PromiseLike;
+ export interface Environment {
+ baseUrl?: string;
+ getWorker?(workerId: string, label: string): Worker;
+ getWorkerUrl?(workerId: string, label: string): string;
+ }
+
export interface IDisposable {
dispose(): void;
}
@@ -43,12 +49,12 @@ declare namespace monaco {
}
declare namespace monaco.editor {
-
+#include(vs/editor/browser/widget/diffNavigator): IDiffNavigator
#includeAll(vs/editor/standalone/browser/standaloneEditor;modes.=>languages.;editorCommon.=>):
#include(vs/editor/standalone/common/standaloneThemeService): BuiltinTheme, IStandaloneThemeData, IColors
#include(vs/editor/common/modes/supports/tokenization): ITokenThemeRule
#include(vs/editor/common/services/webWorker): MonacoWebWorker, IWebWorkerOptions
-#include(vs/editor/standalone/browser/standaloneCodeEditor): IActionDescriptor, IStandaloneEditorConstructionOptions, IDiffEditorConstructionOptions, IStandaloneCodeEditor, IStandaloneDiffEditor
+#include(vs/editor/standalone/browser/standaloneCodeEditor): IActionDescriptor, IGlobalEditorOptions, IStandaloneEditorConstructionOptions, IDiffEditorConstructionOptions, IStandaloneCodeEditor, IStandaloneDiffEditor
export interface ICommandHandler {
(...args: any[]): void;
}
@@ -88,4 +94,4 @@ declare namespace monaco.worker {
}
-//dtsv=2
+//dtsv=3
diff --git a/build/monaco/monaco.usage.recipe b/build/monaco/monaco.usage.recipe
index f1a74a25e3013..3c48da8d85a71 100644
--- a/build/monaco/monaco.usage.recipe
+++ b/build/monaco/monaco.usage.recipe
@@ -2,38 +2,17 @@
// This file is adding references to various symbols which should not be removed via tree shaking
import { ServiceIdentifier } from './vs/platform/instantiation/common/instantiation';
-import { IContextViewService } from './vs/platform/contextview/browser/contextView';
-import { IHighlight } from './vs/base/parts/quickopen/browser/quickOpenModel';
-import { IWorkspaceContextService } from './vs/platform/workspace/common/workspace';
-import { IEnvironmentService } from './vs/platform/environment/common/environment';
-import { CountBadge } from './vs/base/browser/ui/countBadge/countBadge';
-import { SimpleWorkerClient, create as create1 } from './vs/base/common/worker/simpleWorker';
+import { create as create1 } from './vs/base/common/worker/simpleWorker';
import { create as create2 } from './vs/editor/common/services/editorSimpleWorker';
-import { QuickOpenWidget } from './vs/base/parts/quickopen/browser/quickOpenWidget';
-import { WorkbenchAsyncDataTree } from './vs/platform/list/browser/listService';
import { SyncDescriptor0, SyncDescriptor1, SyncDescriptor2, SyncDescriptor3, SyncDescriptor4, SyncDescriptor5, SyncDescriptor6, SyncDescriptor7, SyncDescriptor8 } from './vs/platform/instantiation/common/descriptors';
-import { DiffNavigator } from './vs/editor/browser/widget/diffNavigator';
-import { DocumentRangeFormattingEditProvider } from './vs/editor/common/modes';
import * as editorAPI from './vs/editor/editor.api';
(function () {
var a: any;
var b: any;
- a = (b).layout; // IContextViewProvider
- a = (b).getWorkspaceFolder; // IWorkspaceFolderProvider
- a = (b).getWorkspace; // IWorkspaceFolderProvider
- a = (b).style; // IThemable
- a = (b).style; // IThemable
- a = (>b).style; // IThemable
- a = (b).userHome; // IUserHomeProvider
- a = (b).previous; // IDiffNavigator
a = (>b).type;
- a = (b).start;
- a = (b).end;
- a = (>b).getProxyObject; // IWorkerClient
a = create1;
a = create2;
- a = (b).extensionId;
// injection madness
a = (>b).ctor;
diff --git a/build/monaco/monaco.webpack.config.js b/build/monaco/monaco.webpack.config.js
new file mode 100644
index 0000000000000..974a341a197e1
--- /dev/null
+++ b/build/monaco/monaco.webpack.config.js
@@ -0,0 +1,44 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+const path = require('path');
+
+module.exports = {
+ mode: 'production',
+ entry: {
+ 'core': './build/monaco/esm.core.js',
+ 'editor.worker': './out-monaco-editor-core/esm/vs/editor/editor.worker.js'
+ },
+ output: {
+ globalObject: 'self',
+ filename: '[name].bundle.js',
+ path: path.resolve(__dirname, 'dist')
+ },
+ module: {
+ rules: [{
+ test: /\.css$/,
+ use: ['style-loader', 'css-loader']
+ }, {
+ test: /\.ttf$/,
+ use: ['file-loader']
+ }]
+ },
+ resolve: {
+ alias: {
+ 'monaco-editor-core': path.resolve(__dirname, '../../out-monaco-editor-core/esm/vs/editor/editor.main.js'),
+ }
+ },
+ stats: {
+ all: false,
+ modules: true,
+ maxModules: 0,
+ errors: true,
+ warnings: true,
+ // our additional options
+ moduleTrace: true,
+ errorDetails: true,
+ chunks: true
+ }
+};
diff --git a/build/monaco/package.json b/build/monaco/package.json
index 1ff3e4e72f3fe..70021689eb4a1 100644
--- a/build/monaco/package.json
+++ b/build/monaco/package.json
@@ -1,7 +1,7 @@
{
"name": "monaco-editor-core",
"private": true,
- "version": "0.18.0",
+ "version": "0.19.2",
"description": "A browser based code editor",
"author": "Microsoft Corporation",
"license": "MIT",
diff --git a/build/npm/postinstall.js b/build/npm/postinstall.js
index e89344d9139e5..8f8b0019a7792 100644
--- a/build/npm/postinstall.js
+++ b/build/npm/postinstall.js
@@ -13,7 +13,7 @@ const yarn = process.platform === 'win32' ? 'yarn.cmd' : 'yarn';
* @param {*} [opts]
*/
function yarnInstall(location, opts) {
- opts = opts || {};
+ opts = opts || { env: process.env };
opts.cwd = location;
opts.stdio = 'inherit';
@@ -33,9 +33,10 @@ function yarnInstall(location, opts) {
yarnInstall('extensions'); // node modules shared by all extensions
-yarnInstall('remote'); // node modules used by vscode server
-
-yarnInstall('remote/web'); // node modules used by vscode web
+if (!(process.platform === 'win32' && (process.arch === 'arm64' || process.env['npm_config_arch'] === 'arm64'))) {
+ yarnInstall('remote'); // node modules used by vscode server
+ yarnInstall('remote/web'); // node modules used by vscode web
+}
const allExtensionFolders = fs.readdirSync('extensions');
const extensions = allExtensionFolders.filter(e => {
@@ -52,8 +53,6 @@ extensions.forEach(extension => yarnInstall(`extensions/${extension}`));
function yarnInstallBuildDependencies() {
// make sure we install the deps of build/lib/watch for the system installed
// node, since that is the driver of gulp
- //@ts-ignore
- const env = Object.assign({}, process.env);
const watchPath = path.join(path.dirname(__dirname), 'lib', 'watch');
const yarnrcPath = path.join(watchPath, '.yarnrc');
@@ -66,17 +65,13 @@ target "${target}"
runtime "${runtime}"`;
fs.writeFileSync(yarnrcPath, yarnrc, 'utf8');
- yarnInstall(watchPath, { env });
+ yarnInstall(watchPath);
}
yarnInstall(`build`); // node modules required for build
yarnInstall('test/automation'); // node modules required for smoketest
yarnInstall('test/smoke'); // node modules required for smoketest
+yarnInstall('test/integration/browser'); // node modules required for integration
yarnInstallBuildDependencies(); // node modules for watching, specific to host node version, not electron
-// Remove the windows process tree typings as this causes duplicate identifier errors in tsc builds
-const processTreeDts = path.join('node_modules', 'windows-process-tree', 'typings', 'windows-process-tree.d.ts');
-if (fs.existsSync(processTreeDts)) {
- console.log('Removing windows-process-tree.d.ts');
- fs.unlinkSync(processTreeDts);
-}
+cp.execSync('git config pull.rebase true');
diff --git a/build/npm/preinstall.js b/build/npm/preinstall.js
index eca72654382e0..cb88d37adefd4 100644
--- a/build/npm/preinstall.js
+++ b/build/npm/preinstall.js
@@ -23,7 +23,7 @@ if (majorYarnVersion < 1 || minorYarnVersion < 10) {
err = true;
}
-if (!/yarn\.js$|yarnpkg$/.test(process.env['npm_execpath'])) {
+if (!/yarn[\w-.]*\.js$|yarnpkg$/.test(process.env['npm_execpath'])) {
console.error('\033[1;31m*** Please use yarn to install dependencies.\033[0;0m');
err = true;
}
diff --git a/build/package.json b/build/package.json
index a685a901b88c2..e185594554b75 100644
--- a/build/package.json
+++ b/build/package.json
@@ -6,6 +6,7 @@
"@types/ansi-colors": "^3.2.0",
"@types/azure": "0.9.19",
"@types/debounce": "^1.0.0",
+ "@types/eslint": "4.16.1",
"@types/fancy-log": "^1.3.0",
"@types/glob": "^7.1.1",
"@types/gulp": "^4.0.5",
@@ -28,21 +29,25 @@
"@types/through2": "^2.0.34",
"@types/underscore": "^1.8.9",
"@types/xml2js": "0.0.33",
+ "@typescript-eslint/experimental-utils": "~2.13.0",
+ "@typescript-eslint/parser": "^2.12.0",
"applicationinsights": "1.0.8",
"azure-storage": "^2.1.0",
+ "electron-osx-sign": "^0.4.16",
"github-releases": "^0.4.1",
"gulp-bom": "^1.0.0",
"gulp-sourcemaps": "^1.11.0",
"gulp-uglify": "^3.0.0",
- "iconv-lite": "0.4.23",
+ "iconv-lite-umd": "0.6.8",
+ "jsonc-parser": "^2.3.0",
"mime": "^1.3.4",
- "minimist": "^1.2.0",
+ "minimatch": "3.0.4",
+ "minimist": "^1.2.3",
"request": "^2.85.0",
"terser": "4.3.8",
- "tslint": "^5.9.1",
- "typescript": "3.7.2",
+ "typescript": "^4.1.0-dev.20200824",
"vsce": "1.48.0",
- "vscode-telemetry-extractor": "^1.5.4",
+ "vscode-telemetry-extractor": "^1.6.0",
"xml2js": "^0.4.17"
},
"scripts": {
diff --git a/build/polyfills/vscode-extension-telemetry.js b/build/polyfills/vscode-extension-telemetry.js
new file mode 100644
index 0000000000000..d038776c59c64
--- /dev/null
+++ b/build/polyfills/vscode-extension-telemetry.js
@@ -0,0 +1,26 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+'use strict';
+Object.defineProperty(exports, "__esModule", { value: true });
+
+let TelemetryReporter = (function () {
+ function TelemetryReporter(extensionId, extensionVersion, key) {
+ }
+ TelemetryReporter.prototype.updateUserOptIn = function (key) {
+ };
+ TelemetryReporter.prototype.createAppInsightsClient = function (key) {
+ };
+ TelemetryReporter.prototype.getCommonProperties = function () {
+ };
+ TelemetryReporter.prototype.sendTelemetryEvent = function (eventName, properties, measurements) {
+ };
+ TelemetryReporter.prototype.dispose = function () {
+ };
+ TelemetryReporter.TELEMETRY_CONFIG_ID = 'telemetry';
+ TelemetryReporter.TELEMETRY_CONFIG_ENABLED_ID = 'enableTelemetry';
+ return TelemetryReporter;
+}());
+exports.default = TelemetryReporter;
diff --git a/build/polyfills/vscode-nls.js b/build/polyfills/vscode-nls.js
new file mode 100644
index 0000000000000..b89250102afca
--- /dev/null
+++ b/build/polyfills/vscode-nls.js
@@ -0,0 +1,79 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+'use strict';
+Object.defineProperty(exports, "__esModule", { value: true });
+
+function format(message, args) {
+ let result;
+ // if (isPseudo) {
+ // // FF3B and FF3D is the Unicode zenkaku representation for [ and ]
+ // message = '\uFF3B' + message.replace(/[aouei]/g, '$&$&') + '\uFF3D';
+ // }
+ if (args.length === 0) {
+ result = message;
+ }
+ else {
+ result = message.replace(/\{(\d+)\}/g, function (match, rest) {
+ let index = rest[0];
+ let arg = args[index];
+ let replacement = match;
+ if (typeof arg === 'string') {
+ replacement = arg;
+ }
+ else if (typeof arg === 'number' || typeof arg === 'boolean' || arg === void 0 || arg === null) {
+ replacement = String(arg);
+ }
+ return replacement;
+ });
+ }
+ return result;
+}
+
+function localize(key, message) {
+ let args = [];
+ for (let _i = 2; _i < arguments.length; _i++) {
+ args[_i - 2] = arguments[_i];
+ }
+ return format(message, args);
+}
+
+function loadMessageBundle(file) {
+ return localize;
+}
+
+let MessageFormat;
+(function (MessageFormat) {
+ MessageFormat["file"] = "file";
+ MessageFormat["bundle"] = "bundle";
+ MessageFormat["both"] = "both";
+})(MessageFormat = exports.MessageFormat || (exports.MessageFormat = {}));
+let BundleFormat;
+(function (BundleFormat) {
+ // the nls.bundle format
+ BundleFormat["standalone"] = "standalone";
+ BundleFormat["languagePack"] = "languagePack";
+})(BundleFormat = exports.BundleFormat || (exports.BundleFormat = {}));
+
+exports.loadMessageBundle = loadMessageBundle;
+function config(opts) {
+ if (opts) {
+ if (isString(opts.locale)) {
+ options.locale = opts.locale.toLowerCase();
+ options.language = options.locale;
+ resolvedLanguage = undefined;
+ resolvedBundles = Object.create(null);
+ }
+ if (opts.messageFormat !== undefined) {
+ options.messageFormat = opts.messageFormat;
+ }
+ if (opts.bundleFormat === BundleFormat.standalone && options.languagePackSupport === true) {
+ options.languagePackSupport = false;
+ }
+ }
+ isPseudo = options.locale === 'pseudo';
+ return loadMessageBundle;
+}
+exports.config = config;
diff --git a/build/tsconfig.json b/build/tsconfig.json
index df15ccdd1beb4..f075fe3d4f543 100644
--- a/build/tsconfig.json
+++ b/build/tsconfig.json
@@ -14,7 +14,8 @@
"checkJs": true,
"strict": true,
"noUnusedLocals": true,
- "noUnusedParameters": true
+ "noUnusedParameters": true,
+ "newLine": "lf"
},
"include": [
"**/*.ts"
diff --git a/build/tslint.json b/build/tslint.json
deleted file mode 100644
index 15275279139de..0000000000000
--- a/build/tslint.json
+++ /dev/null
@@ -1,14 +0,0 @@
-{
- "rules": {
- "no-unused-expression": true,
- "no-duplicate-variable": true,
- "curly": true,
- "class-name": true,
- "semicolon": [
- true,
- "always"
- ],
- "triple-equals": true
- },
- "defaultSeverity": "warning"
-}
\ No newline at end of file
diff --git a/build/win32/code.iss b/build/win32/code.iss
index ee70efb974d02..7f4b36e71aabe 100644
--- a/build/win32/code.iss
+++ b/build/win32/code.iss
@@ -1,7 +1,8 @@
+#define RootLicenseFileName FileExists(RepoDir + '\LICENSE.rtf') ? 'LICENSE.rtf' : 'LICENSE.txt'
#define LocalizedLanguageFile(Language = "") \
DirExists(RepoDir + "\licenses") && Language != "" \
? ('; LicenseFile: "' + RepoDir + '\licenses\LICENSE-' + Language + '.rtf"') \
- : '; LicenseFile: "' + RepoDir + '\LICENSE.rtf"'
+ : '; LicenseFile: "' + RepoDir + '\' + RootLicenseFileName + '"'
[Setup]
AppId={#AppId}
@@ -32,6 +33,7 @@ VersionInfoVersion={#RawVersion}
ShowLanguageDialog=auto
ArchitecturesAllowed={#ArchitecturesAllowed}
ArchitecturesInstallIn64BitMode={#ArchitecturesInstallIn64BitMode}
+WizardStyle=modern
#ifdef Sign
SignTool=esrp
@@ -45,7 +47,7 @@ DefaultDirName={pf}\{#DirName}
#endif
[Languages]
-Name: "english"; MessagesFile: "{#RepoDir}\build\win32\i18n\Default.isl,{#RepoDir}\build\win32\i18n\messages.en.isl" {#LocalizedLanguageFile}
+Name: "english"; MessagesFile: "compiler:Default.isl,{#RepoDir}\build\win32\i18n\messages.en.isl" {#LocalizedLanguageFile}
Name: "german"; MessagesFile: "compiler:Languages\German.isl,{#RepoDir}\build\win32\i18n\messages.de.isl" {#LocalizedLanguageFile("deu")}
Name: "spanish"; MessagesFile: "compiler:Languages\Spanish.isl,{#RepoDir}\build\win32\i18n\messages.es.isl" {#LocalizedLanguageFile("esp")}
Name: "french"; MessagesFile: "compiler:Languages\French.isl,{#RepoDir}\build\win32\i18n\messages.fr.isl" {#LocalizedLanguageFile("fra")}
@@ -55,6 +57,9 @@ Name: "russian"; MessagesFile: "compiler:Languages\Russian.isl,{#RepoDir}\build\
Name: "korean"; MessagesFile: "{#RepoDir}\build\win32\i18n\Default.ko.isl,{#RepoDir}\build\win32\i18n\messages.ko.isl" {#LocalizedLanguageFile("kor")}
Name: "simplifiedChinese"; MessagesFile: "{#RepoDir}\build\win32\i18n\Default.zh-cn.isl,{#RepoDir}\build\win32\i18n\messages.zh-cn.isl" {#LocalizedLanguageFile("chs")}
Name: "traditionalChinese"; MessagesFile: "{#RepoDir}\build\win32\i18n\Default.zh-tw.isl,{#RepoDir}\build\win32\i18n\messages.zh-tw.isl" {#LocalizedLanguageFile("cht")}
+Name: "brazilianPortuguese"; MessagesFile: "compiler:Languages\BrazilianPortuguese.isl,{#RepoDir}\build\win32\i18n\messages.pt-br.isl" {#LocalizedLanguageFile("ptb")}
+Name: "hungarian"; MessagesFile: "{#RepoDir}\build\win32\i18n\Default.hu.isl,{#RepoDir}\build\win32\i18n\messages.hu.isl" {#LocalizedLanguageFile("hun")}
+Name: "turkish"; MessagesFile: "compiler:Languages\Turkish.isl,{#RepoDir}\build\win32\i18n\messages.tr.isl" {#LocalizedLanguageFile("trk")}
[InstallDelete]
Type: filesandordirs; Name: "{app}\resources\app\out"; Check: IsNotUpdate
@@ -84,7 +89,7 @@ Source: "{#ProductJsonPath}"; DestDir: "{code:GetDestDir}\resources\app"; Flags:
[Icons]
Name: "{group}\{#NameLong}"; Filename: "{app}\{#ExeBasename}.exe"; AppUserModelID: "{#AppUserId}"
-Name: "{commondesktop}\{#NameLong}"; Filename: "{app}\{#ExeBasename}.exe"; Tasks: desktopicon; AppUserModelID: "{#AppUserId}"
+Name: "{autodesktop}\{#NameLong}"; Filename: "{app}\{#ExeBasename}.exe"; Tasks: desktopicon; AppUserModelID: "{#AppUserId}"
Name: "{userappdata}\Microsoft\Internet Explorer\Quick Launch\{#NameLong}"; Filename: "{app}\{#ExeBasename}.exe"; Tasks: quicklaunchicon; AppUserModelID: "{#AppUserId}"
[Run]
@@ -168,6 +173,13 @@ Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bower
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bowerrc\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\bower.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.bowerrc\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.c++\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.c++\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.c++"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.c++"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,C++}"; Flags: uninsdeletekey; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.c++"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.c++\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\cpp.ico"; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.c++\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
+
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.c\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.c\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.c"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.c"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,C}"; Flags: uninsdeletekey; Tasks: associatewithfiles
@@ -182,6 +194,13 @@ Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cc";
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cc\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\cpp.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cc\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.cjs\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.cjs\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.cjs"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cjs"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,JavaScript}"; Flags: uninsdeletekey; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cjs"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cjs\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\javascript.ico"; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cjs\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
+
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.clj\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.clj\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.clj"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.clj"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Clojure}"; Flags: uninsdeletekey; Tasks: associatewithfiles
@@ -231,6 +250,13 @@ Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.confi
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.config\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\config.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.config\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.containerfile\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.containerfile\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.containerfile"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.containerfile"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,Containerfile}"; Flags: uninsdeletekey; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.containerfile"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.containerfile\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.containerfile\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
+
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.cpp\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.cpp\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.cpp"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.cpp"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,C++}"; Flags: uninsdeletekey; Tasks: associatewithfiles
@@ -423,6 +449,13 @@ Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hbs";
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hbs\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hbs\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.h++\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.h++\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.h++"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.h++"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,C++ Header}"; Flags: uninsdeletekey; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.h++"; ValueType: string; ValueName: "AppUserModelID"; ValueData: "{#AppUserId}"; Flags: uninsdeletekey; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.h++\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\cpp.ico"; Tasks: associatewithfiles
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.h++\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: associatewithfiles
+
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.hh\OpenWithProgids"; ValueType: none; ValueName: "{#RegValueName}"; Flags: deletevalue uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\.hh\OpenWithProgids"; ValueType: string; ValueName: "{#RegValueName}.hh"; ValueData: ""; Flags: uninsdeletevalue; Tasks: associatewithfiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\{#RegValueName}.hh"; ValueType: string; ValueName: ""; ValueData: "{cm:SourceFile,C++ Header}"; Flags: uninsdeletekey; Tasks: associatewithfiles
@@ -957,16 +990,16 @@ Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\Applications\{#ExeBas
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\Applications\{#ExeBasename}.exe\DefaultIcon"; ValueType: string; ValueName: ""; ValueData: "{app}\resources\app\resources\win32\default.ico"
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\Applications\{#ExeBasename}.exe\shell\open\command"; ValueType: string; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""
-Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\*\shell\{#RegValueName}"; ValueType: expandsz; ValueName: ""; ValueData: "Open w&ith {#ShellNameShort}"; Tasks: addcontextmenufiles; Flags: uninsdeletekey
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\*\shell\{#RegValueName}"; ValueType: expandsz; ValueName: ""; ValueData: "{cm:OpenWithCodeContextMenu,{#ShellNameShort}}"; Tasks: addcontextmenufiles; Flags: uninsdeletekey
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\*\shell\{#RegValueName}"; ValueType: expandsz; ValueName: "Icon"; ValueData: "{app}\{#ExeBasename}.exe"; Tasks: addcontextmenufiles
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\*\shell\{#RegValueName}\command"; ValueType: expandsz; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%1"""; Tasks: addcontextmenufiles
-Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\directory\shell\{#RegValueName}"; ValueType: expandsz; ValueName: ""; ValueData: "Open w&ith {#ShellNameShort}"; Tasks: addcontextmenufolders; Flags: uninsdeletekey
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\directory\shell\{#RegValueName}"; ValueType: expandsz; ValueName: ""; ValueData: "{cm:OpenWithCodeContextMenu,{#ShellNameShort}}"; Tasks: addcontextmenufolders; Flags: uninsdeletekey
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\directory\shell\{#RegValueName}"; ValueType: expandsz; ValueName: "Icon"; ValueData: "{app}\{#ExeBasename}.exe"; Tasks: addcontextmenufolders
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\directory\shell\{#RegValueName}\command"; ValueType: expandsz; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%V"""; Tasks: addcontextmenufolders
-Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\directory\background\shell\{#RegValueName}"; ValueType: expandsz; ValueName: ""; ValueData: "Open w&ith {#ShellNameShort}"; Tasks: addcontextmenufolders; Flags: uninsdeletekey
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\directory\background\shell\{#RegValueName}"; ValueType: expandsz; ValueName: ""; ValueData: "{cm:OpenWithCodeContextMenu,{#ShellNameShort}}"; Tasks: addcontextmenufolders; Flags: uninsdeletekey
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\directory\background\shell\{#RegValueName}"; ValueType: expandsz; ValueName: "Icon"; ValueData: "{app}\{#ExeBasename}.exe"; Tasks: addcontextmenufolders
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\directory\background\shell\{#RegValueName}\command"; ValueType: expandsz; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%V"""; Tasks: addcontextmenufolders
-Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\Drive\shell\{#RegValueName}"; ValueType: expandsz; ValueName: ""; ValueData: "Open w&ith {#ShellNameShort}"; Tasks: addcontextmenufolders; Flags: uninsdeletekey
+Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\Drive\shell\{#RegValueName}"; ValueType: expandsz; ValueName: ""; ValueData: "{cm:OpenWithCodeContextMenu,{#ShellNameShort}}"; Tasks: addcontextmenufolders; Flags: uninsdeletekey
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\Drive\shell\{#RegValueName}"; ValueType: expandsz; ValueName: "Icon"; ValueData: "{app}\{#ExeBasename}.exe"; Tasks: addcontextmenufolders
Root: {#SoftwareClassesRootKey}; Subkey: "Software\Classes\Drive\shell\{#RegValueName}\command"; ValueType: expandsz; ValueName: ""; ValueData: """{app}\{#ExeBasename}.exe"" ""%V"""; Tasks: addcontextmenufolders
@@ -996,7 +1029,7 @@ begin
Result := True;
#if "user" == InstallTarget
- if not WizardSilent() and IsAdminLoggedOn() then begin
+ if not WizardSilent() and IsAdmin() then begin
if MsgBox('This User Installer is not meant to be run as an Administrator. If you would like to install VS Code for all users in this system, download the System Installer instead from https://code.visualstudio.com. Are you sure you want to continue?', mbError, MB_OKCANCEL) = IDCANCEL then begin
Result := False;
end;
@@ -1004,7 +1037,7 @@ begin
#endif
#if "user" == InstallTarget
- #if "ia32" == Arch
+ #if "ia32" == Arch || "arm64" == Arch
#define IncompatibleArchRootKey "HKLM32"
#else
#define IncompatibleArchRootKey "HKLM64"
@@ -1114,7 +1147,7 @@ begin
end;
end;
-// http://stackoverflow.com/a/23838239/261019
+// https://stackoverflow.com/a/23838239/261019
procedure Explode(var Dest: TArrayOfString; Text: String; Separator: String);
var
i, p: Integer;
diff --git a/build/win32/i18n/Default.hu.isl b/build/win32/i18n/Default.hu.isl
new file mode 100644
index 0000000000000..8c57d20a59aef
--- /dev/null
+++ b/build/win32/i18n/Default.hu.isl
@@ -0,0 +1,366 @@
+;Inno Setup version 6.0.3+ Hungarian messages
+;Based on the translation of Kornl Pl, kornelpal@gmail.com
+;Istvn Szab, E-mail: istvanszabo890629@gmail.com
+;
+; To download user-contributed translations of this file, go to:
+; http://www.jrsoftware.org/files/istrans/
+;
+; Note: When translating this text, do not add periods (.) to the end of
+; messages that didn't have them already, because on those messages Inno
+; Setup adds the periods automatically (appending a period would result in
+; two periods being displayed).
+
+[LangOptions]
+; The following three entries are very important. Be sure to read and
+; understand the '[LangOptions] section' topic in the help file.
+LanguageName=Magyar
+LanguageID=$040E
+LanguageCodePage=1250
+; If the language you are translating to requires special font faces or
+; sizes, uncomment any of the following entries and change them accordingly.
+;DialogFontName=
+;DialogFontSize=8
+;WelcomeFontName=Verdana
+;WelcomeFontSize=12
+;TitleFontName=Arial CE
+;TitleFontSize=29
+;CopyrightFontName=Arial CE
+;CopyrightFontSize=8
+
+[Messages]
+
+; *** Application titles
+SetupAppTitle=Telept
+SetupWindowTitle=%1 - Telept
+UninstallAppTitle=Eltvolt
+UninstallAppFullTitle=%1 Eltvolt
+
+; *** Misc. common
+InformationTitle=Informcik
+ConfirmTitle=Megerst
+ErrorTitle=Hiba
+
+; *** SetupLdr messages
+SetupLdrStartupMessage=%1 teleptve lesz. Szeretn folytatni?
+LdrCannotCreateTemp=tmeneti fjl ltrehozsa nem lehetsges. A telepts megszaktva
+LdrCannotExecTemp=Fjl futtatsa nem lehetsges az tmeneti knyvtrban. A telepts megszaktva
+HelpTextNote=
+
+; *** Startup error messages
+LastErrorMessage=%1.%n%nHiba %2: %3
+SetupFileMissing=A(z) %1 fjl hinyzik a telept knyvtrbl. Krem hrtsa el a problmt, vagy szerezzen be egy msik pldnyt a programbl!
+SetupFileCorrupt=A teleptsi fjlok srltek. Krem, szerezzen be j msolatot a programbl!
+SetupFileCorruptOrWrongVer=A teleptsi fjlok srltek, vagy inkompatibilisek a telept ezen verzijval. Hrtsa el a problmt, vagy szerezzen be egy msik pldnyt a programbl!
+InvalidParameter=A parancssorba tadott paramter rvnytelen:%n%n%1
+SetupAlreadyRunning=A Telept mr fut.
+WindowsVersionNotSupported=A program nem tmogatja a Windows ezen verzijt.
+WindowsServicePackRequired=A program futtatshoz %1 Service Pack %2 vagy jabb szksges.
+NotOnThisPlatform=Ez a program nem futtathat %1 alatt.
+OnlyOnThisPlatform=Ezt a programot %1 alatt kell futtatni.
+OnlyOnTheseArchitectures=A program kizrlag a kvetkez processzor architektrkhoz tervezett Windows-on telepthet:%n%n%1
+WinVersionTooLowError=A program futtatshoz %1 %2 verzija vagy ksbbi szksges.
+WinVersionTooHighError=Ez a program nem telepthet %1 %2 vagy ksbbire.
+AdminPrivilegesRequired=Csak rendszergazdai mdban telepthet ez a program.
+PowerUserPrivilegesRequired=Csak rendszergazdaknt vagy kiemelt felhasznlknt telepthet ez a program.
+SetupAppRunningError=A telept gy szlelte %1 jelenleg fut.%n%nZrja be az sszes pldnyt, majd kattintson az 'OK'-ra a folytatshoz, vagy a 'Mgse'-re a kilpshez.
+UninstallAppRunningError=Az eltvolt gy szlelte %1 jelenleg fut.%n%nZrja be az sszes pldnyt, majd kattintson az 'OK'-ra a folytatshoz, vagy a 'Mgse'-re a kilpshez.
+
+; *** Startup questions
+PrivilegesRequiredOverrideTitle=Teleptsi md kivlasztsa
+PrivilegesRequiredOverrideInstruction=Vlasszon teleptsi mdot
+PrivilegesRequiredOverrideText1=%1 telepthet az sszes felhasznlnak (rendszergazdai jogok szksgesek), vagy csak magnak.
+PrivilegesRequiredOverrideText2=%1 csak magnak telepthet, vagy az sszes felhasznlnak (rendszergazdai jogok szksgesek).
+PrivilegesRequiredOverrideAllUsers=Telepts &mindenkinek
+PrivilegesRequiredOverrideAllUsersRecommended=Telepts &mindenkinek (ajnlott)
+PrivilegesRequiredOverrideCurrentUser=Telepts csak &nekem
+PrivilegesRequiredOverrideCurrentUserRecommended=Telepts csak &nekem (ajnlott)
+
+; *** Misc. errors
+ErrorCreatingDir=A Telept nem tudta ltrehozni a(z) "%1" knyvtrat
+ErrorTooManyFilesInDir=Nem hozhat ltre fjl a(z) "%1" knyvtrban, mert az mr tl sok fjlt tartalmaz
+
+; *** Setup common messages
+ExitSetupTitle=Kilps a teleptbl
+ExitSetupMessage=A telepts mg folyamatban van. Ha most kilp, a program nem kerl teleptsre.%n%nMsik alkalommal is futtathat a telepts befejezshez%n%nKilp a teleptbl?
+AboutSetupMenuItem=&Nvjegy...
+AboutSetupTitle=Telept nvjegye
+AboutSetupMessage=%1 %2 verzi%n%3%n%nAz %1 honlapja:%n%4
+AboutSetupNote=
+TranslatorNote=
+
+; *** Buttons
+ButtonBack=< &Vissza
+ButtonNext=&Tovbb >
+ButtonInstall=&Telept
+ButtonOK=OK
+ButtonCancel=Mgse
+ButtonYes=&Igen
+ButtonYesToAll=&Mindet
+ButtonNo=&Nem
+ButtonNoToAll=&Egyiket se
+ButtonFinish=&Befejezs
+ButtonBrowse=&Tallzs...
+ButtonWizardBrowse=T&allzs...
+ButtonNewFolder=j &knyvtr
+
+; *** "Select Language" dialog messages
+SelectLanguageTitle=Telept nyelvi bellts
+SelectLanguageLabel=Vlassza ki a telepts alatt hasznlt nyelvet.
+
+; *** Common wizard text
+ClickNext=A folytatshoz kattintson a 'Tovbb'-ra, a kilpshez a 'Mgse'-re.
+BeveledLabel=
+BrowseDialogTitle=Vlasszon knyvtrt
+BrowseDialogLabel=Vlasszon egy knyvtrat az albbi listbl, majd kattintson az 'OK'-ra.
+NewFolderName=j knyvtr
+
+; *** "Welcome" wizard page
+WelcomeLabel1=dvzli a(z) [name] Teleptvarzslja.
+WelcomeLabel2=A(z) [name/ver] teleptsre kerl a szmtgpn.%n%nAjnlott minden, egyb fut alkalmazs bezrsa a folytats eltt.
+
+; *** "Password" wizard page
+WizardPassword=Jelsz
+PasswordLabel1=Ez a telepts jelszval vdett.
+PasswordLabel3=Krem adja meg a jelszt, majd kattintson a 'Tovbb'-ra. A jelszavak kis- s nagy bet rzkenyek lehetnek.
+PasswordEditLabel=&Jelsz:
+IncorrectPassword=Az n ltal megadott jelsz helytelen. Prblja jra.
+
+; *** "License Agreement" wizard page
+WizardLicense=Licencszerzds
+LicenseLabel=Olvassa el figyelmesen az informcikat folytats eltt.
+LicenseLabel3=Krem, olvassa el az albbi licencszerzdst. A telepts folytatshoz, el kell fogadnia a szerzdst.
+LicenseAccepted=&Elfogadom a szerzdst
+LicenseNotAccepted=&Nem fogadom el a szerzdst
+
+; *** "Information" wizard pages
+WizardInfoBefore=Informcik
+InfoBeforeLabel=Olvassa el a kvetkez fontos informcikat a folytats eltt.
+InfoBeforeClickLabel=Ha kszen ll, kattintson a 'Tovbb'-ra.
+WizardInfoAfter=Informcik
+InfoAfterLabel=Olvassa el a kvetkez fontos informcikat a folytats eltt.
+InfoAfterClickLabel=Ha kszen ll, kattintson a 'Tovbb'-ra.
+
+; *** "User Information" wizard page
+WizardUserInfo=Felhasznl adatai
+UserInfoDesc=Krem, adja meg az adatait
+UserInfoName=&Felhasznlnv:
+UserInfoOrg=&Szervezet:
+UserInfoSerial=&Sorozatszm:
+UserInfoNameRequired=Meg kell adnia egy nevet.
+
+; *** "Select Destination Location" wizard page
+WizardSelectDir=Vlasszon clknyvtrat
+SelectDirDesc=Hova telepljn a(z) [name]?
+SelectDirLabel3=A(z) [name] az albbi knyvtrba lesz teleptve.
+SelectDirBrowseLabel=A folytatshoz, kattintson a 'Tovbb'-ra. Ha msik knyvtrat vlasztana, kattintson a 'Tallzs'-ra.
+DiskSpaceGBLabel=At least [gb] GB szabad terletre van szksg.
+DiskSpaceMBLabel=Legalbb [mb] MB szabad terletre van szksg.
+CannotInstallToNetworkDrive=A Telept nem tud hlzati meghajtra telepteni.
+CannotInstallToUNCPath=A Telept nem tud hlzati UNC elrsi tra telepteni.
+InvalidPath=Teljes tvonalat adjon meg, a meghajt betjelvel; pldul:%n%nC:\Alkalmazs%n%nvagy egy hlzati tvonalat a kvetkez alakban:%n%n\\kiszolgl\megoszts
+InvalidDrive=A kivlasztott meghajt vagy hlzati megoszts nem ltezik vagy nem elrhet. Vlasszon egy msikat.
+DiskSpaceWarningTitle=Nincs elg szabad terlet
+DiskSpaceWarning=A Teleptnek legalbb %1 KB szabad lemezterletre van szksge, viszont a kivlasztott meghajtn csupn %2 KB ll rendelkezsre.%n%nMindenkppen folytatja?
+DirNameTooLong=A knyvtr neve vagy az tvonal tl hossz.
+InvalidDirName=A knyvtr neve rvnytelen.
+BadDirName32=A knyvtrak nevei ezen karakterek egyikt sem tartalmazhatjk:%n%n%1
+DirExistsTitle=A knyvtr mr ltezik
+DirExists=A knyvtr:%n%n%1%n%nmr ltezik. Mindenkpp ide akar telepteni?
+DirDoesntExistTitle=A knyvtr nem ltezik
+DirDoesntExist=A knyvtr:%n%n%1%n%nnem ltezik. Szeretn ltrehozni?
+
+; *** "Select Components" wizard page
+WizardSelectComponents=sszetevk kivlasztsa
+SelectComponentsDesc=Mely sszetevk kerljenek teleptsre?
+SelectComponentsLabel2=Jellje ki a teleptend sszetevket; trlje a telepteni nem kvnt sszetevket. Kattintson a 'Tovbb'-ra, ha kszen ll a folytatsra.
+FullInstallation=Teljes telepts
+; if possible don't translate 'Compact' as 'Minimal' (I mean 'Minimal' in your language)
+CompactInstallation=Szoksos telepts
+CustomInstallation=Egyni telepts
+NoUninstallWarningTitle=Ltez sszetev
+NoUninstallWarning=A telept gy tallta, hogy a kvetkez sszetevk mr teleptve vannak a szmtgpre:%n%n%1%n%nEzen sszetevk kijellsnek trlse, nem tvoltja el azokat a szmtgprl.%n%nMindenkppen folytatja?
+ComponentSize1=%1 KB
+ComponentSize2=%1 MB
+ComponentsDiskSpaceMBLabel=A jelenlegi kijells legalbb [gb] GB lemezterletet ignyel.
+ComponentsDiskSpaceMBLabel=A jelenlegi kijells legalbb [mb] MB lemezterletet ignyel.
+
+; *** "Select Additional Tasks" wizard page
+WizardSelectTasks=Tovbbi feladatok
+SelectTasksDesc=Mely kiegszt feladatok kerljenek vgrehajtsra?
+SelectTasksLabel2=Jellje ki, mely kiegszt feladatokat hajtsa vgre a Telept a(z) [name] teleptse sorn, majd kattintson a 'Tovbb'-ra.
+
+; *** "Select Start Menu Folder" wizard page
+WizardSelectProgramGroup=Start Men knyvtra
+SelectStartMenuFolderDesc=Hova helyezze a Telept a program parancsikonjait?
+SelectStartMenuFolderLabel3=A Telept a program parancsikonjait a Start men kvetkez mappjban fogja ltrehozni.
+SelectStartMenuFolderBrowseLabel=A folytatshoz kattintson a 'Tovbb'-ra. Ha msik mappt vlasztana, kattintson a 'Tallzs'-ra.
+MustEnterGroupName=Meg kell adnia egy mappanevet.
+GroupNameTooLong=A knyvtr neve vagy az tvonal tl hossz.
+InvalidGroupName=A knyvtr neve rvnytelen.
+BadGroupName=A knyvtrak nevei ezen karakterek egyikt sem tartalmazhatjk:%n%n%1
+NoProgramGroupCheck2=&Ne hozzon ltre mappt a Start menben
+
+; *** "Ready to Install" wizard page
+WizardReady=Kszen llunk a teleptsre
+ReadyLabel1=A Telept kszen ll, a(z) [name] szmtgpre teleptshez.
+ReadyLabel2a=Kattintson a 'Telepts'-re a folytatshoz, vagy a "Vissza"-ra a belltsok ttekintshez vagy megvltoztatshoz.
+ReadyLabel2b=Kattintson a 'Telepts'-re a folytatshoz.
+ReadyMemoUserInfo=Felhasznl adatai:
+ReadyMemoDir=Telepts clknyvtra:
+ReadyMemoType=Telepts tpusa:
+ReadyMemoComponents=Vlasztott sszetevk:
+ReadyMemoGroup=Start men mappja:
+ReadyMemoTasks=Kiegszt feladatok:
+
+; *** "Preparing to Install" wizard page
+WizardPreparing=Felkszls a teleptsre
+PreparingDesc=A Telept felkszl a(z) [name] szmtgpre trtn teleptshez.
+PreviousInstallNotCompleted=gy korbbi program teleptse/eltvoltsa nem fejezdtt be. jra kell indtania a szmtgpt a msik telepts befejezshez.%n%nA szmtgpe jraindtsa utn ismt futtassa a Teleptt a(z) [name] teleptsnek befejezshez.
+CannotContinue=A telepts nem folytathat. A kilpshez kattintson a 'Mgse'-re
+ApplicationsFound=A kvetkez alkalmazsok olyan fjlokat hasznlnak, amelyeket a Teleptnek frissteni kell. Ajnlott, hogy engedlyezze a Teleptnek ezen alkalmazsok automatikus bezrst.
+ApplicationsFound2=A kvetkez alkalmazsok olyan fjlokat hasznlnak, amelyeket a Teleptnek frissteni kell. Ajnlott, hogy engedlyezze a Teleptnek ezen alkalmazsok automatikus bezrst. A telepts befejezse utn a Telept megksrli az alkalmazsok jraindtst.
+CloseApplications=&Alkalmazsok automatikus bezrsa
+DontCloseApplications=&Ne zrja be az alkalmazsokat
+ErrorCloseApplications=A Telept nem tudott minden alkalmazst automatikusan bezrni. A folytats eltt ajnlott minden, a Telept ltal frisstend fjlokat hasznl alkalmazst bezrni.
+PrepareToInstallNeedsRestart=A teleptnek jra kell indtania a szmtgpet. jraindtst kveten, futtassa jbl a teleptt, a [name] teleptsnek befejezshez .%n%njra szeretn indtani most a szmtgpet?
+
+; *** "Installing" wizard page
+WizardInstalling=Telepts
+InstallingLabel=Krem vrjon, amg a(z) [name] teleptse zajlik.
+
+; *** "Setup Completed" wizard page
+FinishedHeadingLabel=A(z) [name] teleptsnek befejezse
+FinishedLabelNoIcons=A Telept vgzett a(z) [name] teleptsvel.
+FinishedLabel=A Telept vgzett a(z) [name] teleptsvel. Az alkalmazst a ltrehozott ikonok kivlasztsval indthatja.
+ClickFinish=Kattintson a 'Befejezs'-re a kilpshez.
+FinishedRestartLabel=A(z) [name] teleptsnek befejezshez jra kell indtani a szmtgpet. jraindtja most?
+FinishedRestartMessage=A(z) [name] teleptsnek befejezshez, a Teleptnek jra kell indtani a szmtgpet.%n%njraindtja most?
+ShowReadmeCheck=Igen, szeretnm elolvasni a FONTOS fjlt
+YesRadio=&Igen, jraindts most
+NoRadio=&Nem, ksbb indtom jra
+; used for example as 'Run MyProg.exe'
+RunEntryExec=%1 futtatsa
+; used for example as 'View Readme.txt'
+RunEntryShellExec=%1 megtekintse
+
+; *** "Setup Needs the Next Disk" stuff
+ChangeDiskTitle=A Teleptnek szksge van a kvetkez lemezre
+SelectDiskLabel2=Helyezze be a(z) %1. lemezt s kattintson az 'OK'-ra.%n%nHa a fjlok a lemez egy a megjelentettl klnbz mappjban tallhatk, rja be a helyes tvonalat vagy kattintson a 'Tallzs'-ra.
+PathLabel=&tvonal:
+FileNotInDir2=A(z) "%1" fjl nem tallhat a kvetkez helyen: "%2". Helyezze be a megfelel lemezt vagy vlasszon egy msik mappt.
+SelectDirectoryLabel=Adja meg a kvetkez lemez helyt.
+
+; *** Installation phase messages
+SetupAborted=A telepts nem fejezdtt be.%n%nHrtsa el a hibt s futtassa jbl a Teleptt.
+AbortRetryIgnoreSelectAction=Vlasszon mveletet
+AbortRetryIgnoreRetry=&jra
+AbortRetryIgnoreIgnore=&Hiba elvetse s folytats
+AbortRetryIgnoreCancel=Telepts megszaktsa
+
+; *** Installation status messages
+StatusClosingApplications=Alkalmazsok bezrsa...
+StatusCreateDirs=Knyvtrak ltrehozsa...
+StatusExtractFiles=Fjlok kibontsa...
+StatusCreateIcons=Parancsikonok ltrehozsa...
+StatusCreateIniEntries=INI bejegyzsek ltrehozsa...
+StatusCreateRegistryEntries=Rendszerler bejegyzsek ltrehozsa...
+StatusRegisterFiles=Fjlok regisztrlsa...
+StatusSavingUninstall=Eltvolt informcik mentse...
+StatusRunProgram=Telepts befejezse...
+StatusRestartingApplications=Alkalmazsok jraindtsa...
+StatusRollback=Vltoztatsok visszavonsa...
+
+; *** Misc. errors
+ErrorInternal2=Bels hiba: %1
+ErrorFunctionFailedNoCode=Sikertelen %1
+ErrorFunctionFailed=Sikertelen %1; kd: %2
+ErrorFunctionFailedWithMessage=Sikertelen %1; kd: %2.%n%3
+ErrorExecutingProgram=Nem hajthat vgre a fjl:%n%1
+
+; *** Registry errors
+ErrorRegOpenKey=Nem nyithat meg a rendszerler kulcs:%n%1\%2
+ErrorRegCreateKey=Nem hozhat ltre a rendszerler kulcs:%n%1\%2
+ErrorRegWriteKey=Nem mdosthat a rendszerler kulcs:%n%1\%2
+
+; *** INI errors
+ErrorIniEntry=Bejegyzs ltrehozsa sikertelen a kvetkez INI fjlban: "%1".
+
+; *** File copying errors
+FileAbortRetryIgnoreSkipNotRecommended=&Fjl kihagysa (nem ajnlott)
+FileAbortRetryIgnoreIgnoreNotRecommended=&Hiba elvetse s folytats (nem ajnlott)
+SourceIsCorrupted=A forrsfjl megsrlt
+SourceDoesntExist=A(z) "%1" forrsfjl nem ltezik
+ExistingFileReadOnly2=A fjl csak olvashatknt van jellve.
+ExistingFileReadOnlyRetry=Csak &olvashat tulajdonsg eltvoltsa s jra prblkozs
+ExistingFileReadOnlyKeepExisting=&Ltez fjl megtartsa
+ErrorReadingExistingDest=Hiba lpett fel a fjl olvassa kzben:
+FileExists=A fjl mr ltezik.%n%nFell kvnja rni?
+ExistingFileNewer=A ltez fjl jabb a teleptsre kerlnl. Ajnlott a ltez fjl megtartsa.%n%nMeg kvnja tartani a ltez fjlt?
+ErrorChangingAttr=Hiba lpett fel a fjl attribtumnak mdostsa kzben:
+ErrorCreatingTemp=Hiba lpett fel a fjl teleptsi knyvtrban trtn ltrehozsa kzben:
+ErrorReadingSource=Hiba lpett fel a forrsfjl olvassa kzben:
+ErrorCopying=Hiba lpett fel a fjl msolsa kzben:
+ErrorReplacingExistingFile=Hiba lpett fel a ltez fjl cserje kzben:
+ErrorRestartReplace=A fjl cserje az jraindts utn sikertelen volt:
+ErrorRenamingTemp=Hiba lpett fel fjl teleptsi knyvtrban trtn tnevezse kzben:
+ErrorRegisterServer=Nem lehet regisztrlni a DLL-t/OCX-et: %1
+ErrorRegSvr32Failed=Sikertelen RegSvr32. A visszaadott kd: %1
+ErrorRegisterTypeLib=Nem lehet regisztrlni a tpustrat: %1
+
+; *** Uninstall display name markings
+; used for example as 'My Program (32-bit)'
+UninstallDisplayNameMark=%1 (%2)
+; used for example as 'My Program (32-bit, All users)'
+UninstallDisplayNameMarks=%1 (%2, %3)
+UninstallDisplayNameMark32Bit=32-bit
+UninstallDisplayNameMark64Bit=64-bit
+UninstallDisplayNameMarkAllUsers=Minden felhasznl
+UninstallDisplayNameMarkCurrentUser=Jelenlegi felhasznl
+
+; *** Post-installation errors
+ErrorOpeningReadme=Hiba lpett fel a FONTOS fjl megnyitsa kzben.
+ErrorRestartingComputer=A Telept nem tudta jraindtani a szmtgpet. Indtsa jra kzileg.
+
+; *** Uninstaller messages
+UninstallNotFound=A(z) "%1" fjl nem ltezik. Nem tvolthat el.
+UninstallOpenError=A(z) "%1" fjl nem nyithat meg. Nem tvolthat el.
+UninstallUnsupportedVer=A(z) "%1" eltvoltsi naplfjl formtumt nem tudja felismerni az eltvolt jelen verzija. Az eltvolts nem folytathat
+UninstallUnknownEntry=Egy ismeretlen bejegyzs (%1) tallhat az eltvoltsi naplfjlban
+ConfirmUninstall=Biztosan el kvnja tvoltani a(z) %1 programot s minden sszetevjt?
+UninstallOnlyOnWin64=Ezt a teleptst csak 64-bites Windowson lehet eltvoltani.
+OnlyAdminCanUninstall=Ezt a teleptst csak adminisztrcis jogokkal rendelkez felhasznl tvolthatja el.
+UninstallStatusLabel=Legyen trelemmel, amg a(z) %1 szmtgprl trtn eltvoltsa befejezdik.
+UninstalledAll=A(z) %1 sikeresen el lett tvoltva a szmtgprl.
+UninstalledMost=A(z) %1 eltvoltsa befejezdtt.%n%nNhny elemet nem lehetett eltvoltani. Trlje kzileg.
+UninstalledAndNeedsRestart=A(z) %1 eltvoltsnak befejezshez jra kell indtania a szmtgpt.%n%njraindtja most?
+UninstallDataCorrupted=A(z) "%1" fjl srlt. Nem tvolthat el.
+
+; *** Uninstallation phase messages
+ConfirmDeleteSharedFileTitle=Trli a megosztott fjlt?
+ConfirmDeleteSharedFile2=A rendszer azt jelzi, hogy a kvetkez megosztott fjlra mr nincs szksge egyetlen programnak sem. Eltvoltja a megosztott fjlt?%n%nHa ms programok mg mindig hasznljk a megosztott fjlt, akkor az eltvoltsa utn lehet, hogy nem fognak megfelelen mkdni. Ha bizonytalan, vlassza a Nemet. A fjl megtartsa nem okoz problmt a rendszerben.
+SharedFileNameLabel=Fjlnv:
+SharedFileLocationLabel=Helye:
+WizardUninstalling=Eltvolts llapota
+StatusUninstalling=%1 eltvoltsa...
+
+; *** Shutdown block reasons
+ShutdownBlockReasonInstallingApp=%1 teleptse.
+ShutdownBlockReasonUninstallingApp=%1 eltvoltsa.
+
+; The custom messages below aren't used by Setup itself, but if you make
+; use of them in your scripts, you'll want to translate them.
+
+[CustomMessages]
+
+NameAndVersion=%1, verzi: %2
+AdditionalIcons=Tovbbi parancsikonok:
+CreateDesktopIcon=&Asztali ikon ltrehozsa
+CreateQuickLaunchIcon=&Gyorsindt parancsikon ltrehozsa
+ProgramOnTheWeb=%1 az interneten
+UninstallProgram=Eltvolts - %1
+LaunchProgram=Indts %1
+AssocFileExtension=A(z) %1 &trstsa a(z) %2 fjlkiterjesztssel
+AssocingFileExtension=A(z) %1 trstsa a(z) %2 fjlkiterjesztssel...
+AutoStartProgramGroupDescription=Indtpult:
+AutoStartProgram=%1 automatikus indtsa
+AddonHostProgramNotFound=A(z) %1 nem tallhat a kivlasztott knyvtrban.%n%nMindenkppen folytatja?
diff --git a/build/win32/i18n/Default.isl b/build/win32/i18n/Default.isl
deleted file mode 100644
index 370da6b37c712..0000000000000
--- a/build/win32/i18n/Default.isl
+++ /dev/null
@@ -1,336 +0,0 @@
-; *** Inno Setup version 5.5.3+ English messages ***
-;
-; To download user-contributed translations of this file, go to:
-; http://www.jrsoftware.org/files/istrans/
-;
-; Note: When translating this text, do not add periods (.) to the end of
-; messages that didn't have them already, because on those messages Inno
-; Setup adds the periods automatically (appending a period would result in
-; two periods being displayed).
-
-[LangOptions]
-; The following three entries are very important. Be sure to read and
-; understand the '[LangOptions] section' topic in the help file.
-LanguageName=English
-LanguageID=$0409
-LanguageCodePage=0
-; If the language you are translating to requires special font faces or
-; sizes, uncomment any of the following entries and change them accordingly.
-;DialogFontName=
-;DialogFontSize=8
-;WelcomeFontName=Verdana
-;WelcomeFontSize=12
-;TitleFontName=Arial
-;TitleFontSize=29
-;CopyrightFontName=Arial
-;CopyrightFontSize=8
-
-[Messages]
-
-; *** Application titles
-SetupAppTitle=Setup
-SetupWindowTitle=Setup - %1
-UninstallAppTitle=Uninstall
-UninstallAppFullTitle=%1 Uninstall
-
-; *** Misc. common
-InformationTitle=Information
-ConfirmTitle=Confirm
-ErrorTitle=Error
-
-; *** SetupLdr messages
-SetupLdrStartupMessage=This will install %1. Do you wish to continue?
-LdrCannotCreateTemp=Unable to create a temporary file. Setup aborted
-LdrCannotExecTemp=Unable to execute file in the temporary directory. Setup aborted
-
-; *** Startup error messages
-LastErrorMessage=%1.%n%nError %2: %3
-SetupFileMissing=The file %1 is missing from the installation directory. Please correct the problem or obtain a new copy of the program.
-SetupFileCorrupt=The setup files are corrupted. Please obtain a new copy of the program.
-SetupFileCorruptOrWrongVer=The setup files are corrupted, or are incompatible with this version of Setup. Please correct the problem or obtain a new copy of the program.
-InvalidParameter=An invalid parameter was passed on the command line:%n%n%1
-SetupAlreadyRunning=Setup is already running.
-WindowsVersionNotSupported=This program does not support the version of Windows your computer is running.
-WindowsServicePackRequired=This program requires %1 Service Pack %2 or later.
-NotOnThisPlatform=This program will not run on %1.
-OnlyOnThisPlatform=This program must be run on %1.
-OnlyOnTheseArchitectures=This program can only be installed on versions of Windows designed for the following processor architectures:%n%n%1
-MissingWOW64APIs=The version of Windows you are running does not include functionality required by Setup to perform a 64-bit installation. To correct this problem, please install Service Pack %1.
-WinVersionTooLowError=This program requires %1 version %2 or later.
-WinVersionTooHighError=This program cannot be installed on %1 version %2 or later.
-AdminPrivilegesRequired=You must be logged in as an administrator when installing this program.
-PowerUserPrivilegesRequired=You must be logged in as an administrator or as a member of the Power Users group when installing this program.
-SetupAppRunningError=Setup has detected that %1 is currently running.%n%nPlease close all instances of it now, then click OK to continue, or Cancel to exit.
-UninstallAppRunningError=Uninstall has detected that %1 is currently running.%n%nPlease close all instances of it now, then click OK to continue, or Cancel to exit.
-
-; *** Misc. errors
-ErrorCreatingDir=Setup was unable to create the directory "%1"
-ErrorTooManyFilesInDir=Unable to create a file in the directory "%1" because it contains too many files
-
-; *** Setup common messages
-ExitSetupTitle=Exit Setup
-ExitSetupMessage=Setup is not complete. If you exit now, the program will not be installed.%n%nYou may run Setup again at another time to complete the installation.%n%nExit Setup?
-AboutSetupMenuItem=&About Setup...
-AboutSetupTitle=About Setup
-AboutSetupMessage=%1 version %2%n%3%n%n%1 home page:%n%4
-AboutSetupNote=
-TranslatorNote=
-
-; *** Buttons
-ButtonBack=< &Back
-ButtonNext=&Next >
-ButtonInstall=&Install
-ButtonOK=OK
-ButtonCancel=Cancel
-ButtonYes=&Yes
-ButtonYesToAll=Yes to &All
-ButtonNo=&No
-ButtonNoToAll=N&o to All
-ButtonFinish=&Finish
-ButtonBrowse=&Browse...
-ButtonWizardBrowse=B&rowse...
-ButtonNewFolder=&Make New Folder
-
-; *** "Select Language" dialog messages
-SelectLanguageTitle=Select Setup Language
-SelectLanguageLabel=Select the language to use during the installation:
-
-; *** Common wizard text
-ClickNext=Click Next to continue, or Cancel to exit Setup.
-BeveledLabel=
-BrowseDialogTitle=Browse For Folder
-BrowseDialogLabel=Select a folder in the list below, then click OK.
-NewFolderName=New Folder
-
-; *** "Welcome" wizard page
-WelcomeLabel1=Welcome to the [name] Setup Wizard
-WelcomeLabel2=This will install [name/ver] on your computer.%n%nIt is recommended that you close all other applications before continuing.
-
-; *** "Password" wizard page
-WizardPassword=Password
-PasswordLabel1=This installation is password protected.
-PasswordLabel3=Please provide the password, then click Next to continue. Passwords are case-sensitive.
-PasswordEditLabel=&Password:
-IncorrectPassword=The password you entered is not correct. Please try again.
-
-; *** "License Agreement" wizard page
-WizardLicense=License Agreement
-LicenseLabel=Please read the following important information before continuing.
-LicenseLabel3=Please read the following License Agreement. You must accept the terms of this agreement before continuing with the installation.
-LicenseAccepted=I &accept the agreement
-LicenseNotAccepted=I &do not accept the agreement
-
-; *** "Information" wizard pages
-WizardInfoBefore=Information
-InfoBeforeLabel=Please read the following important information before continuing.
-InfoBeforeClickLabel=When you are ready to continue with Setup, click Next.
-WizardInfoAfter=Information
-InfoAfterLabel=Please read the following important information before continuing.
-InfoAfterClickLabel=When you are ready to continue with Setup, click Next.
-
-; *** "User Information" wizard page
-WizardUserInfo=User Information
-UserInfoDesc=Please enter your information.
-UserInfoName=&User Name:
-UserInfoOrg=&Organization:
-UserInfoSerial=&Serial Number:
-UserInfoNameRequired=You must enter a name.
-
-; *** "Select Destination Location" wizard page
-WizardSelectDir=Select Destination Location
-SelectDirDesc=Where should [name] be installed?
-SelectDirLabel3=Setup will install [name] into the following folder.
-SelectDirBrowseLabel=To continue, click Next. If you would like to select a different folder, click Browse.
-DiskSpaceMBLabel=At least [mb] MB of free disk space is required.
-CannotInstallToNetworkDrive=Setup cannot install to a network drive.
-CannotInstallToUNCPath=Setup cannot install to a UNC path.
-InvalidPath=You must enter a full path with drive letter; for example:%n%nC:\APP%n%nor a UNC path in the form:%n%n\\server\share
-InvalidDrive=The drive or UNC share you selected does not exist or is not accessible. Please select another.
-DiskSpaceWarningTitle=Not Enough Disk Space
-DiskSpaceWarning=Setup requires at least %1 KB of free space to install, but the selected drive only has %2 KB available.%n%nDo you want to continue anyway?
-DirNameTooLong=The folder name or path is too long.
-InvalidDirName=The folder name is not valid.
-BadDirName32=Folder names cannot include any of the following characters:%n%n%1
-DirExistsTitle=Folder Exists
-DirExists=The folder:%n%n%1%n%nalready exists. Would you like to install to that folder anyway?
-DirDoesntExistTitle=Folder Does Not Exist
-DirDoesntExist=The folder:%n%n%1%n%ndoes not exist. Would you like the folder to be created?
-
-; *** "Select Components" wizard page
-WizardSelectComponents=Select Components
-SelectComponentsDesc=Which components should be installed?
-SelectComponentsLabel2=Select the components you want to install; clear the components you do not want to install. Click Next when you are ready to continue.
-FullInstallation=Full installation
-; if possible don't translate 'Compact' as 'Minimal' (I mean 'Minimal' in your language)
-CompactInstallation=Compact installation
-CustomInstallation=Custom installation
-NoUninstallWarningTitle=Components Exist
-NoUninstallWarning=Setup has detected that the following components are already installed on your computer:%n%n%1%n%nDeselecting these components will not uninstall them.%n%nWould you like to continue anyway?
-ComponentSize1=%1 KB
-ComponentSize2=%1 MB
-ComponentsDiskSpaceMBLabel=Current selection requires at least [mb] MB of disk space.
-
-; *** "Select Additional Tasks" wizard page
-WizardSelectTasks=Select Additional Tasks
-SelectTasksDesc=Which additional tasks should be performed?
-SelectTasksLabel2=Select the additional tasks you would like Setup to perform while installing [name], then click Next.
-
-; *** "Select Start Menu Folder" wizard page
-WizardSelectProgramGroup=Select Start Menu Folder
-SelectStartMenuFolderDesc=Where should Setup place the program's shortcuts?
-SelectStartMenuFolderLabel3=Setup will create the program's shortcuts in the following Start Menu folder.
-SelectStartMenuFolderBrowseLabel=To continue, click Next. If you would like to select a different folder, click Browse.
-MustEnterGroupName=You must enter a folder name.
-GroupNameTooLong=The folder name or path is too long.
-InvalidGroupName=The folder name is not valid.
-BadGroupName=The folder name cannot include any of the following characters:%n%n%1
-NoProgramGroupCheck2=&Don't create a Start Menu folder
-
-; *** "Ready to Install" wizard page
-WizardReady=Ready to Install
-ReadyLabel1=Setup is now ready to begin installing [name] on your computer.
-ReadyLabel2a=Click Install to continue with the installation, or click Back if you want to review or change any settings.
-ReadyLabel2b=Click Install to continue with the installation.
-ReadyMemoUserInfo=User information:
-ReadyMemoDir=Destination location:
-ReadyMemoType=Setup type:
-ReadyMemoComponents=Selected components:
-ReadyMemoGroup=Start Menu folder:
-ReadyMemoTasks=Additional tasks:
-
-; *** "Preparing to Install" wizard page
-WizardPreparing=Preparing to Install
-PreparingDesc=Setup is preparing to install [name] on your computer.
-PreviousInstallNotCompleted=The installation/removal of a previous program was not completed. You will need to restart your computer to complete that installation.%n%nAfter restarting your computer, run Setup again to complete the installation of [name].
-CannotContinue=Setup cannot continue. Please click Cancel to exit.
-ApplicationsFound=The following applications are using files that need to be updated by Setup. It is recommended that you allow Setup to automatically close these applications.
-ApplicationsFound2=The following applications are using files that need to be updated by Setup. It is recommended that you allow Setup to automatically close these applications. After the installation has completed, Setup will attempt to restart the applications.
-CloseApplications=&Automatically close the applications
-DontCloseApplications=&Do not close the applications
-ErrorCloseApplications=Setup was unable to automatically close all applications. It is recommended that you close all applications using files that need to be updated by Setup before continuing.
-
-; *** "Installing" wizard page
-WizardInstalling=Installing
-InstallingLabel=Please wait while Setup installs [name] on your computer.
-
-; *** "Setup Completed" wizard page
-FinishedHeadingLabel=Completing the [name] Setup Wizard
-FinishedLabelNoIcons=Setup has finished installing [name] on your computer.
-FinishedLabel=Setup has finished installing [name] on your computer. The application may be launched by selecting the installed icons.
-ClickFinish=Click Finish to exit Setup.
-FinishedRestartLabel=To complete the installation of [name], Setup must restart your computer. Would you like to restart now?
-FinishedRestartMessage=To complete the installation of [name], Setup must restart your computer.%n%nWould you like to restart now?
-ShowReadmeCheck=Yes, I would like to view the README file
-YesRadio=&Yes, restart the computer now
-NoRadio=&No, I will restart the computer later
-; used for example as 'Run MyProg.exe'
-RunEntryExec=Run %1
-; used for example as 'View Readme.txt'
-RunEntryShellExec=View %1
-
-; *** "Setup Needs the Next Disk" stuff
-ChangeDiskTitle=Setup Needs the Next Disk
-SelectDiskLabel2=Please insert Disk %1 and click OK.%n%nIf the files on this disk can be found in a folder other than the one displayed below, enter the correct path or click Browse.
-PathLabel=&Path:
-FileNotInDir2=The file "%1" could not be located in "%2". Please insert the correct disk or select another folder.
-SelectDirectoryLabel=Please specify the location of the next disk.
-
-; *** Installation phase messages
-SetupAborted=Setup was not completed.%n%nPlease correct the problem and run Setup again.
-EntryAbortRetryIgnore=Click Retry to try again, Ignore to proceed anyway, or Abort to cancel installation.
-
-; *** Installation status messages
-StatusClosingApplications=Closing applications...
-StatusCreateDirs=Creating directories...
-StatusExtractFiles=Extracting files...
-StatusCreateIcons=Creating shortcuts...
-StatusCreateIniEntries=Creating INI entries...
-StatusCreateRegistryEntries=Creating registry entries...
-StatusRegisterFiles=Registering files...
-StatusSavingUninstall=Saving uninstall information...
-StatusRunProgram=Finishing installation...
-StatusRestartingApplications=Restarting applications...
-StatusRollback=Rolling back changes...
-
-; *** Misc. errors
-ErrorInternal2=Internal error: %1
-ErrorFunctionFailedNoCode=%1 failed
-ErrorFunctionFailed=%1 failed; code %2
-ErrorFunctionFailedWithMessage=%1 failed; code %2.%n%3
-ErrorExecutingProgram=Unable to execute file:%n%1
-
-; *** Registry errors
-ErrorRegOpenKey=Error opening registry key:%n%1\%2
-ErrorRegCreateKey=Error creating registry key:%n%1\%2
-ErrorRegWriteKey=Error writing to registry key:%n%1\%2
-
-; *** INI errors
-ErrorIniEntry=Error creating INI entry in file "%1".
-
-; *** File copying errors
-FileAbortRetryIgnore=Click Retry to try again, Ignore to skip this file (not recommended), or Abort to cancel installation.
-FileAbortRetryIgnore2=Click Retry to try again, Ignore to proceed anyway (not recommended), or Abort to cancel installation.
-SourceIsCorrupted=The source file is corrupted
-SourceDoesntExist=The source file "%1" does not exist
-ExistingFileReadOnly=The existing file is marked as read-only.%n%nClick Retry to remove the read-only attribute and try again, Ignore to skip this file, or Abort to cancel installation.
-ErrorReadingExistingDest=An error occurred while trying to read the existing file:
-FileExists=The file already exists.%n%nWould you like Setup to overwrite it?
-ExistingFileNewer=The existing file is newer than the one Setup is trying to install. It is recommended that you keep the existing file.%n%nDo you want to keep the existing file?
-ErrorChangingAttr=An error occurred while trying to change the attributes of the existing file:
-ErrorCreatingTemp=An error occurred while trying to create a file in the destination directory:
-ErrorReadingSource=An error occurred while trying to read the source file:
-ErrorCopying=An error occurred while trying to copy a file:
-ErrorReplacingExistingFile=An error occurred while trying to replace the existing file:
-ErrorRestartReplace=RestartReplace failed:
-ErrorRenamingTemp=An error occurred while trying to rename a file in the destination directory:
-ErrorRegisterServer=Unable to register the DLL/OCX: %1
-ErrorRegSvr32Failed=RegSvr32 failed with exit code %1
-ErrorRegisterTypeLib=Unable to register the type library: %1
-
-; *** Post-installation errors
-ErrorOpeningReadme=An error occurred while trying to open the README file.
-ErrorRestartingComputer=Setup was unable to restart the computer. Please do this manually.
-
-; *** Uninstaller messages
-UninstallNotFound=File "%1" does not exist. Cannot uninstall.
-UninstallOpenError=File "%1" could not be opened. Cannot uninstall
-UninstallUnsupportedVer=The uninstall log file "%1" is in a format not recognized by this version of the uninstaller. Cannot uninstall
-UninstallUnknownEntry=An unknown entry (%1) was encountered in the uninstall log
-ConfirmUninstall=Are you sure you want to completely remove %1? Extensions and settings will not be removed.
-UninstallOnlyOnWin64=This installation can only be uninstalled on 64-bit Windows.
-OnlyAdminCanUninstall=This installation can only be uninstalled by a user with administrative privileges.
-UninstallStatusLabel=Please wait while %1 is removed from your computer.
-UninstalledAll=%1 was successfully removed from your computer.
-UninstalledMost=%1 uninstall complete.%n%nSome elements could not be removed. These can be removed manually.
-UninstalledAndNeedsRestart=To complete the uninstallation of %1, your computer must be restarted.%n%nWould you like to restart now?
-UninstallDataCorrupted="%1" file is corrupted. Cannot uninstall
-
-; *** Uninstallation phase messages
-ConfirmDeleteSharedFileTitle=Remove Shared File?
-ConfirmDeleteSharedFile2=The system indicates that the following shared file is no longer in use by any programs. Would you like for Uninstall to remove this shared file?%n%nIf any programs are still using this file and it is removed, those programs may not function properly. If you are unsure, choose No. Leaving the file on your system will not cause any harm.
-SharedFileNameLabel=File name:
-SharedFileLocationLabel=Location:
-WizardUninstalling=Uninstall Status
-StatusUninstalling=Uninstalling %1...
-
-; *** Shutdown block reasons
-ShutdownBlockReasonInstallingApp=Installing %1.
-ShutdownBlockReasonUninstallingApp=Uninstalling %1.
-
-; The custom messages below aren't used by Setup itself, but if you make
-; use of them in your scripts, you'll want to translate them.
-
-[CustomMessages]
-
-NameAndVersion=%1 version %2
-AdditionalIcons=Additional icons:
-CreateDesktopIcon=Create a &desktop icon
-CreateQuickLaunchIcon=Create a &Quick Launch icon
-ProgramOnTheWeb=%1 on the Web
-UninstallProgram=Uninstall %1
-LaunchProgram=Launch %1
-AssocFileExtension=&Associate %1 with the %2 file extension
-AssocingFileExtension=Associating %1 with the %2 file extension...
-AutoStartProgramGroupDescription=Startup:
-AutoStartProgram=Automatically start %1
-AddonHostProgramNotFound=%1 could not be located in the folder you selected.%n%nDo you want to continue anyway?
diff --git a/build/win32/i18n/Default.ko.isl b/build/win32/i18n/Default.ko.isl
index a7c38d12b9f90..0f1b1a7ccf521 100644
--- a/build/win32/i18n/Default.ko.isl
+++ b/build/win32/i18n/Default.ko.isl
@@ -1,12 +1,16 @@
-; *** Inno Setup version 5.5.3+ Korean messages ***
-;
-; To download user-contributed translations of this file, go to:
-; http://www.jrsoftware.org/files/istrans/
+; *** Inno Setup version 6.0.0+ Korean messages ***
;
+; 6.0.3+ Translator: SungDong Kim (acroedit@gmail.com)
+; 5.5.3+ Translator: Domddol (domddol@gmail.com)
+; Translation date: MAR 04, 2014
+; Contributors: Hansoo KIM (iryna7@gmail.com), Woong-Jae An (a183393@hanmail.net)
+; Storage: http://www.jrsoftware.org/files/istrans/
+; ο ѱ Ģ ؼմϴ.
; Note: When translating this text, do not add periods (.) to the end of
; messages that didn't have them already, because on those messages Inno
; Setup adds the periods automatically (appending a period would result in
; two periods being displayed).
+
[LangOptions]
; The following three entries are very important. Be sure to read and
; understand the '[LangOptions] section' topic in the help file.
@@ -23,50 +27,68 @@ LanguageCodePage=949
;TitleFontSize=29
;CopyrightFontName=Arial
;CopyrightFontSize=8
+
[Messages]
+
; *** Application titles
SetupAppTitle=ġ
-SetupWindowTitle=ġ - %1
+SetupWindowTitle=%1 ġ
UninstallAppTitle=
UninstallAppFullTitle=%1
+
; *** Misc. common
InformationTitle=
ConfirmTitle=Ȯ
ErrorTitle=
+
; *** SetupLdr messages
-SetupLdrStartupMessage= %1() ġ˴ϴ. Ͻðڽϱ?
-LdrCannotCreateTemp=ӽ ϴ. ġ α ߴܵǾϴ.
-LdrCannotExecTemp=ӽ ϴ. ġ α ߴܵǾϴ.
+SetupLdrStartupMessage=%1() ġմϴ, Ͻðڽϱ?
+LdrCannotCreateTemp=ӽ ϴ, ġ ߴմϴ
+LdrCannotExecTemp=ӽ ϴ, ġ ߴմϴ
+HelpTextNote=
+
; *** Startup error messages
LastErrorMessage=%1.%n%n %2: %3
-SetupFileMissing= %1() ġ Ǿϴ. ذϰų α .
-SetupFileCorrupt=ġ ջǾϴ. α .
-SetupFileCorruptOrWrongVer=ġ ջǾų ġ α ȣȯ ʽϴ. ذϰų α .
-InvalidParameter=ٿ ߸ Ű :%n%n%1
-SetupAlreadyRunning=ġ α ̹ Դϴ.
-WindowsVersionNotSupported= α ǻͿ Windows ʽϴ.
-WindowsServicePackRequired= α ġϷ %1 %2 ̻ ʿմϴ.
-NotOnThisPlatform= α %1 ʽϴ.
+SetupFileMissing=%1 ʽϴ, ذ ų ο ġ α Ͻñ ٶϴ.
+SetupFileCorrupt=ġ ջǾϴ, ο ġ α Ͻñ ٶϴ.
+SetupFileCorruptOrWrongVer=ġ ջ̰ų ġ ȣȯ ʽϴ, ذ ų ο ġ α Ͻñ ٶϴ.
+InvalidParameter=߸ Ű Դϴ:%n%n%1
+SetupAlreadyRunning=ġ ̹ Դϴ.
+WindowsVersionNotSupported= α Windows ʽϴ.
+WindowsServicePackRequired= α Ϸ %1 sp%2 ̻̾ մϴ.
+NotOnThisPlatform= α %1 ۵ ʽϴ.
OnlyOnThisPlatform= α %1 ؾ մϴ.
-OnlyOnTheseArchitectures= α μ Űó %n%n%1 Windows ġ ֽϴ.
-MissingWOW64APIs= Windows ġ α 64Ʈ ġϴ ʿ ϴ. ذϷ %1() ġϼ.
-WinVersionTooLowError= α ġϷ %1 %2 ̻ ʿմϴ.
-WinVersionTooHighError= α %1 %2 ̻ ġ ϴ.
-AdminPrivilegesRequired= α ġ ڷ αؾ մϴ.
-PowerUserPrivilegesRequired= α ġ ڳ αؾ մϴ.
-SetupAppRunningError=ġ α %1() ߽ϴ.%n%n νϽ ݰ Ϸ [Ȯ], Ϸ [] Ŭϼ.
-UninstallAppRunningError= ۾ %1() ߽ϴ.%n%n νϽ ݰ Ϸ [Ȯ], Ϸ [] Ŭϼ.
+OnlyOnTheseArchitectures= α Ʒ ó ȣȯǴ Windows ġ ֽϴ:%n%n%1
+WinVersionTooLowError= α %1 %2 ̻ ʿմϴ.
+WinVersionTooHighError= α %1 %2 ̻ ġ ϴ.
+AdminPrivilegesRequired= α ġϷ ڷ αؾ մϴ.
+PowerUserPrivilegesRequired= α ġϷ Ǵ ڷ αؾ մϴ.
+SetupAppRunningError= %1() Դϴ!%n%n װ νϽ ݾ ֽʽÿ. Ϸ "Ȯ", Ϸ "" ŬϽʽÿ.
+UninstallAppRunningError= %1() Դϴ!%n%n װ νϽ ݾ ֽʽÿ. Ϸ "Ȯ", Ϸ "" ŬϽʽÿ.
+
+; *** Startup questions
+PrivilegesRequiredOverrideTitle=ġ
+PrivilegesRequiredOverrideInstruction=ġ 带 ֽʽÿ
+PrivilegesRequiredOverrideText1=%1 ( ʿ) Ǵ ڿ ġմϴ.
+PrivilegesRequiredOverrideText2=%1 Ǵ ( ʿ) ġմϴ.
+PrivilegesRequiredOverrideAllUsers= ڿ ġ(&A)
+PrivilegesRequiredOverrideAllUsersRecommended= ڿ ġ(&A) (õ)
+PrivilegesRequiredOverrideCurrentUser= ڿ ġ(&M)
+PrivilegesRequiredOverrideCurrentUserRecommended= ڿ ġ(&M) (õ)
+
; *** Misc. errors
-ErrorCreatingDir=ġ α "%1"() ϴ.
-ErrorTooManyFilesInDir= "%1" ʹ Ƿ ϴ.
+ErrorCreatingDir="%1" ϴ.
+ErrorTooManyFilesInDir="%1" ʹ ϴ.
+
; *** Setup common messages
-ExitSetupTitle=ġ
-ExitSetupMessage=ġ Ϸ ʾҽϴ. ϸ α ġ ʽϴ.%n%n߿ ġ α ٽ Ͽ ġ ֽϴ.%n%nġ α Ͻðڽϱ?
-AboutSetupMenuItem=ġ α (&A)...
-AboutSetupTitle=ġ α
-AboutSetupMessage=%1 %2%n%3%n%n%1 Ȩ:%n%4
+ExitSetupTitle=ġ Ϸ
+ExitSetupMessage=ġ Ϸ ʾҽϴ, ⼭ ġ ϸ α ġ ʽϴ.%n%nġ ϷϷ ߿ ٽ ġ α ؾ մϴ.%n%n ġ Ͻðڽϱ?
+AboutSetupMenuItem=ġ (&A)...
+AboutSetupTitle=ġ
+AboutSetupMessage=%1 %2%n%3%n%n%1 Ȩ :%n%4
AboutSetupNote=
TranslatorNote=
+
; *** Buttons
ButtonBack=< ڷ(&B)
ButtonNext=(&N) >
@@ -75,224 +97,271 @@ ButtonOK=Ȯ
ButtonCancel=
ButtonYes=(&Y)
ButtonYesToAll= (&A)
-ButtonNo=ƴϿ(&N)
-ButtonNoToAll= ƴϿ(&O)
-ButtonFinish=ħ(&F)
+ButtonNo=ƴϿ(&N)
+ButtonNoToAll= ƴϿ(&O)
+ButtonFinish=(&F)
ButtonBrowse=ãƺ(&B)...
-ButtonWizardBrowse=ãƺ(&R)
+ButtonWizardBrowse=ãƺ(&R)...
ButtonNewFolder= (&M)
+
; *** "Select Language" dialog messages
SelectLanguageTitle=ġ
-SelectLanguageLabel=ġ ߿ ϼ.
+SelectLanguageLabel=ġ Ͻʽÿ.
+
; *** Common wizard text
-ClickNext=Ϸ [] Ŭϰ ġ α Ϸ [] Ŭϼ.
+ClickNext=Ϸ "" Ŭϰ ġ Ϸ "" Ŭմϴ.
BeveledLabel=
BrowseDialogTitle= ãƺ
-BrowseDialogLabel=Ʒ Ͽ [Ȯ] Ŭϼ.
+BrowseDialogLabel=Ʒ Ͽ "Ȯ" Ŭմϴ.
NewFolderName=
+
; *** "Welcome" wizard page
WelcomeLabel1=[name] ġ
-WelcomeLabel2= ǻͿ [name/ver]() ġմϴ.%n%nϱ ٸ α ݴ ϴ.
+WelcomeLabel2= ǻͿ [name/ver]() ġ Դϴ.%n%nġϱ ٸ α ñ ٶϴ.
+
; *** "Password" wizard page
-WizardPassword=ȣ
-PasswordLabel1= ġ ȣ ȣǰ ֽϴ.
-PasswordLabel3=Ϸ ȣ Է [] Ŭϼ. ȣ ҹڸ մϴ.
-PasswordEditLabel=ȣ(&P):
-IncorrectPassword=Է ȣ ߸Ǿϴ. ٽ õϼ.
+WizardPassword= ȣ
+PasswordLabel1= ġ ȣ ȣǾ ֽϴ.
+PasswordLabel3= ȣ Էϰ "" ŬϽʽÿ. ȣ ҹڸ ؾ մϴ.
+PasswordEditLabel= ȣ(&P):
+IncorrectPassword= ȣ Ȯ ʽϴ, ٽ ԷϽʽÿ.
+
; *** "License Agreement" wizard page
WizardLicense=
-LicenseLabel=ϱ ߿ о .
-LicenseLabel3= о ּ. ġ Ϸ ǿ ؾ մϴ.
-LicenseAccepted= (&A)
-LicenseNotAccepted= (&D)
+LicenseLabel=ϱ ߿ оʽÿ.
+LicenseLabel3= оʽÿ, ġ Ϸ ؾ մϴ.
+LicenseAccepted=մϴ(&A)
+LicenseNotAccepted= ʽϴ(&D)
+
; *** "Information" wizard pages
WizardInfoBefore=
-InfoBeforeLabel=ϱ ߿ о .
-InfoBeforeClickLabel=ġ غ Ǹ [] Ŭմϴ.
+InfoBeforeLabel=ϱ ߿ оʽÿ.
+InfoBeforeClickLabel=ġ Ϸ "" ŬϽʽÿ.
WizardInfoAfter=
-InfoAfterLabel=ϱ ߿ о .
-InfoAfterClickLabel=ġ غ Ǹ [] Ŭմϴ.
+InfoAfterLabel=ϱ ߿ оʽÿ.
+InfoAfterClickLabel=ġ Ϸ "" ŬϽʽÿ.
+
; *** "User Information" wizard page
WizardUserInfo=
-UserInfoDesc= Էϼ.
+UserInfoDesc= ԷϽʽÿ.
UserInfoName= ̸(&U):
UserInfoOrg=(&O):
-UserInfoSerial=Ϸ ȣ(&S):
-UserInfoNameRequired≠ Էؾ մϴ.
+UserInfoSerial=ø ȣ(&S):
+UserInfoNameRequired= ̸ ԷϽʽÿ.
+
; *** "Select Destination Location" wizard page
-WizardSelectDir= ġ
-SelectDirDesc=[name]() ġϽðڽϱ?
-SelectDirLabel3=ġ α [name]() ġմϴ.
-SelectDirBrowseLabel=Ϸ [] Ŭϼ. ٸ Ϸ [ãƺ] Ŭϼ.
-DiskSpaceMBLabel= [mb]MB ũ ʿմϴ.
-CannotInstallToNetworkDrive=ġ α Ʈũ ̺꿡 ġ ϴ.
-CannotInstallToUNCPath=ġ α UNC ο ġ ϴ.
-InvalidPath=̺ ڿ Բ ü θ Էؾ մϴ. :%n%nC:\APP%n%nǴ UNC :%n%n\\server\share
-InvalidDrive= ̺곪 UNC ų ϴ. ٸ ̺곪 UNC ϼ.
-DiskSpaceWarningTitle=ũ
-DiskSpaceWarning=ġ α ġϷ ġ %1KB ʿ ̺ %2KBۿ ϴ.%n%n Ͻðڽϱ?
+WizardSelectDir=ġ ġ
+SelectDirDesc=[name] ġ ġ Ͻʽÿ.
+SelectDirLabel3= [name]() ġմϴ.
+SelectDirBrowseLabel=Ϸ "", ٸ Ϸ "ãƺ" ŬϽʽÿ.
+DiskSpaceGBLabel= α ּ [gb] GB ũ ʿմϴ.
+DiskSpaceMBLabel= α ּ [mb] MB ũ ʿմϴ.
+CannotInstallToNetworkDrive=Ʈũ ̺꿡 ġ ϴ.
+CannotInstallToUNCPath=UNC ο ġ ϴ.
+InvalidPath=̺ ڸ ü θ ԷϽʽÿ.%n : C:\APP %n%nǴ, UNC θ ԷϽʽÿ.%n : \\server\share
+InvalidDrive= ̺ Ǵ UNC ʰų ϴ, ٸ θ Ͻʽÿ.
+DiskSpaceWarningTitle=ũ մϴ
+DiskSpaceWarning=ġ ּ %1 KB ũ ʿ, ̺ %2 KB ۿ ϴ.%n%n Ͻðڽϱ?
DirNameTooLong= ̸ Ǵ ΰ ʹ ϴ.
-InvalidDirName= ̸ ߸Ǿϴ.
-BadDirName32= ̸ %n%n%1 ڸ ϴ.
-DirExistsTitle=
-DirExists= %n%n%1%n%n() ̹ ֽϴ. ش ġϽðڽϱ?
-DirDoesntExistTitle=
-DirDoesntExist= %n%n%1%n%n() ϴ. ðڽϱ?
+InvalidDirName= ̸ ȿ ʽϴ.
+BadDirName32= ̸ ڸ ϴ:%n%n%1
+DirExistsTitle= մϴ
+DirExists= %n%n%1%n%n() ̹ մϴ, ġϽðڽϱ?
+DirDoesntExistTitle= ʽϴ
+DirDoesntExist= %n%n%1%n%n() ʽϴ, ðڽϱ?
+
; *** "Select Components" wizard page
WizardSelectComponents=
-SelectComponentsDesc= Ҹ ġϽðڽϱ?
-SelectComponentsLabel2=ġ Ҵ ϰ ġ Ҵ 켼. غ Ǹ [] Ŭϼ.
-FullInstallation=ü ġ
+SelectComponentsDesc=ġ Ҹ Ͻʽÿ.
+SelectComponentsLabel2=ʿ Ҵ üũϰ ʿ Ҵ üũ մϴ, Ϸ "" ŬϽʽÿ.
+FullInstallation= ġ
; if possible don't translate 'Compact' as 'Minimal' (I mean 'Minimal' in your language)
-CompactInstallation=Compact ġ
+CompactInstallation=ּ ġ
CustomInstallation= ġ
-NoUninstallWarningTitle= Ұ
-NoUninstallWarning=ġ α %n%n%1%n%n() ǻͿ ̹ ġǾ ߽ϴ. ̷ Ҵ ص ŵ ʽϴ.%n%n Ͻðڽϱ?
-ComponentSize1=%1KB
-ComponentSize2=%1MB
-ComponentsDiskSpaceMBLabel= ؼ [mb]MB ũ ʿմϴ.
+NoUninstallWarningTitle= Ұ մϴ
+NoUninstallWarning= Ұ ̹ ġǾ ֽϴ:%n%n%1%n%n , α Ž ҵ ŵ ̴ϴ.%n%n Ͻðڽϱ?
+ComponentSize1=%1 KB
+ComponentSize2=%1 MB
+ComponentsDiskSpaceGBLabel= ּ [gb] GB ũ ʿմϴ.
+ComponentsDiskSpaceMBLabel= ּ [mb] MB ũ ʿմϴ.
+
; *** "Select Additional Tasks" wizard page
WizardSelectTasks=߰ ۾
-SelectTasksDesc= ۾ ߰ Ͻðڽϱ?
-SelectTasksLabel2=ġ α [name]() ġϴ ߰ ۾ [] Ŭϼ.
+SelectTasksDesc= ߰ ۾ Ͻʽÿ.
+SelectTasksLabel2=[name] ġ ߰ ۾ , "" ŬϽʽÿ.
+
; *** "Select Start Menu Folder" wizard page
WizardSelectProgramGroup=
-SelectStartMenuFolderDesc=ġ α α ٷ ⸦ 鵵 Ͻðڽϱ?
-SelectStartMenuFolderLabel3=ġ α α ٷ ⸦ ϴ.
-SelectStartMenuFolderBrowseLabel=Ϸ [] Ŭϼ. ٸ Ϸ [ãƺ] Ŭϼ.
-MustEnterGroupName= ̸ Էؾ մϴ.
+SelectStartMenuFolderDesc= α ٷΰ⸦ ġϰڽϱ?
+SelectStartMenuFolderLabel3= α ٷΰ⸦ ϴ.
+SelectStartMenuFolderBrowseLabel=Ϸ "" Ŭϰ, ٸ Ϸ "ãƺ" ŬϽʽÿ.
+MustEnterGroupName= ̸ ԷϽʽÿ.
GroupNameTooLong= ̸ Ǵ ΰ ʹ ϴ.
-InvalidGroupName= ̸ ߸Ǿϴ.
-BadGroupName= ̸ %n%n%1 ڸ ϴ.
+InvalidGroupName= ̸ ȿ ʽϴ.
+BadGroupName= ̸ ڸ ϴ:%n%n%1
NoProgramGroupCheck2= (&D)
+
; *** "Ready to Install" wizard page
-WizardReady=ġ غ
-ReadyLabel1= ġ α ǻͿ [name] ġ غ Ǿϴ.
-ReadyLabel2a=ġ Ϸ [ġ] Ŭϰ, ϰų Ϸ [ڷ] Ŭϼ.
-ReadyLabel2b=ġ Ϸ [ġ] Ŭϼ.
+WizardReady=ġ غ Ϸ
+ReadyLabel1= ǻͿ [name]() ġ غ Ǿϴ.
+ReadyLabel2a=ġ Ϸ "ġ", ϰų Ϸ "ڷ" ŬϽʽÿ.
+ReadyLabel2b=ġ Ϸ "ġ" ŬϽʽÿ.
ReadyMemoUserInfo= :
-ReadyMemoDir= ġ:
+ReadyMemoDir=ġ ġ:
ReadyMemoType=ġ :
ReadyMemoComponents= :
ReadyMemoGroup= :
ReadyMemoTasks=߰ ۾:
+
; *** "Preparing to Install" wizard page
WizardPreparing=ġ غ
-PreparingDesc=ġ α ǻͿ [name] ġ غϰ ֽϴ.
-PreviousInstallNotCompleted= α ġ/ ۾ Ϸ ʾҽϴ. ش ġ ϷϷ ǻ ٽ ؾ մϴ.%n%nǻ ٽ [name] ġ ϷϷ ġ α ٽ ϼ.
-CannotContinue=ġ α ϴ. Ϸ [] Ŭϼ.
-ApplicationsFound=ġ α Ʈؾ ϴ α ǰ ֽϴ. ġ α ̷ α ڵ ݵ ϴ ϴ.
-ApplicationsFound2=ġ α Ʈؾ ϴ α ǰ ֽϴ. ġ α ̷ α ڵ ݵ ϴ ϴ. ġ ϷǸ ġ α α ٽ Ϸ õմϴ.
-CloseApplications= α ڵ ݱ(&A)
-DontCloseApplications= α (&D)
-ErrorCloseApplications=ġ α Ϻ α ڵ ϴ. ϱ ġ α Ʈؾ ϴ ϴ α ݴ ϴ.
+PreparingDesc= ǻͿ [name] ġ غϴ Դϴ.
+PreviousInstallNotCompleted= α ġ/ ۾ Ϸ ʾҽϴ, ϷϷ ǻ ٽ ؾ մϴ.%n%nǻ ٽ , ġ 縦 ٽ Ͽ [name] ġ ϷϽñ ٶϴ.
+CannotContinue=ġ ϴ, "" ŬϿ ġ Ͻʽÿ.
+ApplicationsFound= α ġ Ʈ ʿ ϰ ֽϴ, ġ 簡 ̷ α ڵ ֵ Ͻñ ٶϴ.
+ApplicationsFound2= α ġ Ʈ ʿ ϰ ֽϴ, ġ 簡 ̷ α ڵ ֵ Ͻñ ٶϴ. ġ ϷǸ, ġ α ٽ ۵ǵ õ ̴ϴ.
+CloseApplications=ڵ α (&A)
+DontCloseApplications=α (&D)
+ErrorCloseApplications=ġ 簡 α ڵ ϴ, ϱ ġ Ʈ ʿ ϰ ִ α Ͻñ ٶϴ.
+PrepareToInstallNeedsRestart=ġ ǻ ؾ մϴ. [name] ġ Ϸϱ ǻ ٽ Ŀ ġ 縦 ٽ ֽʽÿ.%n%n ٽ Ͻðڽϱ?
+
; *** "Installing" wizard page
WizardInstalling=ġ
-InstallingLabel=ġ α ǻͿ [name]() ġϴ ٷ ּ.
+InstallingLabel= ǻͿ [name]() ġϴ ... ٷ ֽʽÿ.
+
; *** "Setup Completed" wizard page
-FinishedHeadingLabel=[name] 縦 Ϸϴ
-FinishedLabelNoIcons=ġ α ǻͿ [name]() ġ߽ϴ.
-FinishedLabel=ġ α ǻͿ [name]() ġ߽ϴ. ġ ٷ ⸦ Ͽ ش α ֽϴ.
-ClickFinish=ġ α Ϸ [ħ] Ŭϼ.
-FinishedRestartLabel=[name] ġ ϷϷ ġ α ǻ ٽ ؾ մϴ. ٽ Ͻðڽϱ?
-FinishedRestartMessage=[name] ġ ϷϷ ġ α ǻ ٽ ؾ մϴ.%n%n ٽ Ͻðڽϱ?
-ShowReadmeCheck=, README ڽϴ.
-YesRadio=, ǻ ٽ ϰڽϴ(&Y).
-NoRadio=ƴϿ, ǻ ߿ ٽ ϰڽϴ(&N).
+FinishedHeadingLabel=[name] ġ Ϸ
+FinishedLabelNoIcons= ǻͿ [name]() ġǾϴ.
+FinishedLabel= ǻͿ [name]() ġǾϴ, α ġ Ͽ ֽϴ.
+ClickFinish=ġ "" ŬϽʽÿ.
+FinishedRestartLabel=[name] ġ ϷϷ, ǻ ٽ ؾ մϴ. ٽ Ͻðڽϱ?
+FinishedRestartMessage=[name] ġ ϷϷ, ǻ ٽ ؾ մϴ.%n%n ٽ Ͻðڽϱ?
+ShowReadmeCheck=, README ǥմϴ
+YesRadio=, ٽ մϴ(&Y)
+NoRadio=ƴϿ, ߿ ٽ մϴ(&N)
; used for example as 'Run MyProg.exe'
RunEntryExec=%1
; used for example as 'View Readme.txt'
-RunEntryShellExec=%1
+RunEntryShellExec=%1 ǥ
+
; *** "Setup Needs the Next Disk" stuff
-ChangeDiskTitle=ġ α ũ ʿ
-SelectDiskLabel2=ũ %1() [Ȯ] Ŭϼ.%n%n ũ Ʒ ǥõ ƴ ٸ ùٸ θ Էϰų [ãƺ] Ŭϼ.
+ChangeDiskTitle=ũ ʿմϴ
+SelectDiskLabel2=ũ %1() ϰ "Ȯ" ŬϽʽÿ.%n%n ũ Ʒ ΰ ƴ ִ , ùٸ θ Էϰų "ãƺ" ŬϽñ ٶϴ.
PathLabel=(&P):
-FileNotInDir2="%2" "%1"() ã ϴ. ùٸ ũ ϰų ٸ ϼ.
-SelectDirectoryLabel= ũ ġ ϼ.
+FileNotInDir2=%2 %1() ġ ϴ, ùٸ ũ ϰų ٸ Ͻʽÿ.
+SelectDirectoryLabel= ũ ġ Ͻʽÿ.
+
; *** Installation phase messages
-SetupAborted=ġ Ϸ ߽ϴ.%n%n ذ ġ α ٽ ϼ.
-EntryAbortRetryIgnore=ٽ õϷ [ٽ õ], Ϸ [], ġ Ϸ [ߴ] Ŭϼ.
+SetupAborted=ġ Ϸ ʾҽϴ.%n%n ذ , ٽ ġ Ͻʽÿ.
+AbortRetryIgnoreSelectAction= ֽʽÿ.
+AbortRetryIgnoreRetry=õ(&T)
+AbortRetryIgnoreIgnore= ϰ (&I)
+AbortRetryIgnoreCancel=ġ
+
; *** Installation status messages
-StatusClosingApplications= α ݴ ...
-StatusCreateDirs= ...
+StatusClosingApplications=α ϴ ...
+StatusCreateDirs= ...
StatusExtractFiles= ϴ ...
-StatusCreateIcons=ٷ ⸦ ...
+StatusCreateIcons=ٷΰ⸦ ϴ ...
StatusCreateIniEntries=INI ...
StatusCreateRegistryEntries=Ʈ ...
StatusRegisterFiles= ϴ ...
StatusSavingUninstall= ϴ ...
StatusRunProgram=ġ Ϸϴ ...
-StatusRestartingApplications= α ٽ ϴ ...
-StatusRollback= ѹϴ ...
+StatusRestartingApplications=α ٽ ϴ ...
+StatusRollback= ϴ ...
+
; *** Misc. errors
ErrorInternal2= : %1
ErrorFunctionFailedNoCode=%1
-ErrorFunctionFailed=%1 , ڵ %2
-ErrorFunctionFailedWithMessage=%1 , ڵ %2.%n%3
-ErrorExecutingProgram= :%n%1
+ErrorFunctionFailed=%1 ; ڵ %2
+ErrorFunctionFailedWithMessage=%1 , ڵ: %2.%n%3
+ErrorExecutingProgram= :%n%1
+
; *** Registry errors
-ErrorRegOpenKey=Ʈ Ű :%n%1\%2
-ErrorRegCreateKey=Ʈ Ű :%n%1\%2
-ErrorRegWriteKey=Ʈ Ű ϴ :%n%1\%2
+ErrorRegOpenKey=Ʈ Ű :%n%1\%2
+ErrorRegCreateKey=Ʈ Ű :%n%1\%2
+ErrorRegWriteKey=Ʈ Ű :%n%1\%2
+
; *** INI errors
-ErrorIniEntry= "%1" INI ߿ ߽ϴ.
+ErrorIniEntry=%1 Ͽ INI Դϴ.
+
; *** File copying errors
-FileAbortRetryIgnore=ٽ õϷ [ٽ õ], dzʶٷ []( ), ġ Ϸ [ߴ] Ŭϼ.
-FileAbortRetryIgnore2=ٽ õϷ [ٽ õ], Ϸ []( ), ġ Ϸ [ߴ] Ŭϼ.
-SourceIsCorrupted= ջǾϴ.
-SourceDoesntExist= "%1"() ϴ.
-ExistingFileReadOnly= б ǥõǾ ֽϴ.%n%nб Ư ϰ ٽ õϷ [ٽ õ], dzʶٷ [], ġ Ϸ [ߴ] Ŭϼ.
-ErrorReadingExistingDest= д :
-FileExists=ش ̹ ֽϴ.%n%nġ α Ͻðڽϱ?
-ExistingFileNewer= ġ α ġϷ Ϻ ֽԴϴ. մϴ.%n%n Ͻðڽϱ?
-ErrorChangingAttr= Ư ϴ :
-ErrorCreatingTemp= :
-ErrorReadingSource= д :
-ErrorCopying= ϴ :
-ErrorReplacingExistingFile= ٲٴ :
+FileAbortRetryIgnoreSkipNotRecommended= dzʶ(&S) ( ʽϴ)
+FileAbortRetryIgnoreIgnoreNotRecommended= ϰ (&I) ( ʽϴ)
+SourceIsCorrupted= ջ
+SourceDoesntExist= %1()
+ExistingFileReadOnly2= б ̱ ü ϴ.
+ExistingFileReadOnlyRetry=б Ӽ ϰ ٽ õϷ(&R)
+ExistingFileReadOnlyKeepExisting= (&K)
+ErrorReadingExistingDest= д :
+FileExists= ̹ մϴ.%n%n ðڽϱ?
+ExistingFileNewer= ġϷ ϴ Ϻ Դϴ, Ͻñ ٶϴ.%n%n Ͻðڽϱ?
+ErrorChangingAttr= Ӽ ϴ :
+ErrorCreatingTemp= :
+ErrorReadingSource= д :
+ErrorCopying= ϴ :
+ErrorReplacingExistingFile= üϴ :
ErrorRestartReplace=RestartReplace :
-ErrorRenamingTemp= ִ ̸ ٲٴ :
-ErrorRegisterServer=DLL/OCX : %1
-ErrorRegSvr32Failed= ڵ %1() Բ RegSvr32
-ErrorRegisterTypeLib= ̺귯 : %1
+ErrorRenamingTemp= ̸ ٲٴ :
+ErrorRegisterServer=DLL/OCX : %1
+ErrorRegSvr32Failed=RegSvr32 ڵ : %1
+ErrorRegisterTypeLib= ̺귯 Ͽ : %1
+
+; *** Uninstall display name markings
+; used for example as 'My Program (32-bit)'
+UninstallDisplayNameMark=%1 (%2)
+; used for example as 'My Program (32-bit, All users)'
+UninstallDisplayNameMarks=%1 (%2, %3)
+UninstallDisplayNameMark32Bit=32Ʈ
+UninstallDisplayNameMark64Bit=64Ʈ
+UninstallDisplayNameMarkAllUsers=
+UninstallDisplayNameMarkCurrentUser=
+
; *** Post-installation errors
-ErrorOpeningReadme=README ߿ ߽ϴ.
-ErrorRestartingComputer=ġ α ǻ ٽ ϴ. ϼ.
+ErrorOpeningReadme=README ߽ϴ.
+ErrorRestartingComputer=ǻ ٽ ϴ, ٽ Ͻʽÿ.
+
; *** Uninstaller messages
-UninstallNotFound= "%1"() ϴ. ϴ.
-UninstallOpenError= "%1"() ϴ. ϴ.
-UninstallUnsupportedVer= α "%1"() α ν ϴ Դϴ. ϴ.
-UninstallUnknownEntry= α (%1) ߰ߵǾϴ.
-ConfirmUninstall=%1() ش Ҹ Ͻðڽϱ?
-UninstallOnlyOnWin64= ġ 64Ʈ Windows ֽϴ.
-OnlyAdminCanUninstall= ġ ִ ڸ ֽϴ.
-UninstallStatusLabel=ǻͿ %1() ϴ ٷ ּ.
-UninstalledAll=ǻͿ %1() ߽ϴ.
-UninstalledMost=%1 Ű ϷǾϴ.%n%nϺ Ҵ ϴ. ̷ ֽϴ.
-UninstalledAndNeedsRestart=%1 Ÿ ϷϷ ǻ ٽ ؾ մϴ.%n%n ٽ Ͻðڽϱ?
-UninstallDataCorrupted="%1" ջǾϴ. ϴ.
+UninstallNotFound= %1() ʱ , Ÿ ϴ.
+UninstallOpenError= %1() , Ÿ ϴ.
+UninstallUnsupportedVer= α "%1"() ν ̱ , Ÿ ϴ.
+UninstallUnknownEntry= %1() α ԵǾ ֽϴ.
+ConfirmUninstall= %1() Ҹ Ͻðڽϱ?
+UninstallOnlyOnWin64= α 64Ʈ Windows ֽϴ.
+OnlyAdminCanUninstall= α Ϸ ʿմϴ.
+UninstallStatusLabel= ǻͿ %1() ϴ ... ٷ ֽʽÿ.
+UninstalledAll=%1() ŵǾϴ!
+UninstalledMost=%1 Ű ϷǾϴ.%n%nϺ Ҵ , Ͻñ ٶϴ.
+UninstalledAndNeedsRestart=%1 Ÿ ϷϷ, ǻ ٽ ؾ մϴ.%n%n ٽ Ͻðڽϱ?
+UninstallDataCorrupted= "%1"() ջǾ , Ÿ ϴ.
+
; *** Uninstallation phase messages
ConfirmDeleteSharedFileTitle= Ͻðڽϱ?
-ConfirmDeleteSharedFile2=ýۿ ϴ α ǥõ˴ϴ. ۾ Ͻðڽϱ?%n%n ϴ α ִµ ϸ ش α ùٸ ۵ ֽϴ. [ƴϿ] ϼ. ýۿ ״ ξ ƹ ʽϴ.
+ConfirmDeleteSharedFile2=ý α ʽϴ, Ͻðڽϱ?%n%n ٸ α ϰ ִ ¿ , ش α ۵ , Ȯ "ƴϿ" ϼŵ ˴ϴ. ýۿ ־ ʽϴ.
SharedFileNameLabel= ̸:
SharedFileLocationLabel=ġ:
WizardUninstalling=
StatusUninstalling=%1() ϴ ...
+
; *** Shutdown block reasons
ShutdownBlockReasonInstallingApp=%1() ġϴ Դϴ.
ShutdownBlockReasonUninstallingApp=%1() ϴ Դϴ.
+
; The custom messages below aren't used by Setup itself, but if you make
; use of them in your scripts, you'll want to translate them.
+
[CustomMessages]
+
NameAndVersion=%1 %2
-AdditionalIcons=߰ ٷ :
-CreateDesktopIcon= ȭ ٷ (&D)
-CreateQuickLaunchIcon= ٷ (&Q)
-ProgramOnTheWeb=%1
+AdditionalIcons= ߰:
+CreateDesktopIcon= ȭ鿡 ٷΰ (&D)
+CreateQuickLaunchIcon= (&Q)
+ProgramOnTheWeb=%1
UninstallProgram=%1
-LaunchProgram=%1
-AssocFileExtension=%1() %2 Ȯ (&A)
-AssocingFileExtension=%1() %2 Ȯ ...
+LaunchProgram=%1
+AssocFileExtension= Ȯ %2() %1 մϴ.
+AssocingFileExtension= Ȯ %2() %1 ϴ ...
AutoStartProgramGroupDescription=:
-AutoStartProgram=%1 ڵ
-AddonHostProgramNotFound= %1() ã ϴ.%n%n Ͻðڽϱ?
\ No newline at end of file
+AutoStartProgram=%1() ڵ
+AddonHostProgramNotFound=%1() ġ ϴ.%n%n Ͻðڽϱ?
diff --git a/build/win32/i18n/Default.zh-cn.isl b/build/win32/i18n/Default.zh-cn.isl
index e384e83d30d36..5c5df9a166f0d 100644
--- a/build/win32/i18n/Default.zh-cn.isl
+++ b/build/win32/i18n/Default.zh-cn.isl
@@ -1,16 +1,17 @@
-; *** Inno Setup version 5.5.3+ Simplified Chinese messages ***
+; *** Inno Setup version 6.0.3+ Chinese Simplified messages ***
;
-; To download user-contributed translations of this file, go to:
-; http://www.jrsoftware.org/files/istrans/
+; Maintained by Zhenghan Yang
+; Email: 847320916@QQ.com
+; Translation based on network resource
+; The latest Translation is on https://github.com/kira-96/Inno-Setup-Chinese-Simplified-Translation
;
-; Note: When translating this text, do not add periods (.) to the end of
-; messages that didn't have them already, because on those messages Inno
-; Setup adds the periods automatically (appending a period would result in
-; two periods being displayed).
+
[LangOptions]
; The following three entries are very important. Be sure to read and
; understand the '[LangOptions] section' topic in the help file.
-LanguageName=Simplified Chinese
+LanguageName=简体中文
+; If Language Name display incorrect, uncomment next line
+; LanguageName=<7B80><4F53><4E2D><6587>
LanguageID=$0804
LanguageCodePage=936
; If the language you are translating to requires special font faces or
@@ -23,276 +24,342 @@ LanguageCodePage=936
;TitleFontSize=29
;CopyrightFontName=Arial
;CopyrightFontSize=8
+
[Messages]
-; *** Application titles
-SetupAppTitle=װ
-SetupWindowTitle=װ - %1
-UninstallAppTitle=ж
-UninstallAppFullTitle=%1 ж
+
+; *** 应用程序标题
+SetupAppTitle=安装
+SetupWindowTitle=安装 - %1
+UninstallAppTitle=卸载
+UninstallAppFullTitle=%1 卸载
+
; *** Misc. common
-InformationTitle=Ϣ
-ConfirmTitle=ȷ
-ErrorTitle=
+InformationTitle=信息
+ConfirmTitle=确认
+ErrorTitle=错误
+
; *** SetupLdr messages
-SetupLdrStartupMessage=⽫װ %1ǷҪ?
-LdrCannotCreateTemp=ʱļװֹ
-LdrCannotExecTemp=ʱĿ¼ִļװֹ
-; *** Startup error messages
-LastErrorMessage=%1%n%n %2: %3
-SetupFileMissing=װĿ¼ȱʧļ %1ȡ¸
-SetupFileCorrupt=װļȡó¸
-SetupFileCorruptOrWrongVer=װļ˰װ汾ݡȡó¸
-InvalidParameter= %n%n%1 ϴһЧ
-SetupAlreadyRunning=װС
-WindowsVersionNotSupported=˳֧е Windows 汾
-WindowsServicePackRequired=˳Ҫ %1 %2 ߰汾
-NotOnThisPlatform=˳ %1 С
-OnlyOnThisPlatform=˳ %1 С
-OnlyOnTheseArchitectures=˳ɰװΪ´ϵṹƵ Windows 汾:%n%n%1
-MissingWOW64APIs=е Windows 汾װִ 64 λװĹܡҪ⣬밲װ %1
-WinVersionTooLowError=˳Ҫ %1 汾 %2 ߰汾
-WinVersionTooHighError=˳ܰװ %1 汾 %2 ߵİ汾ϡ
-AdminPrivilegesRequired=ڰװ˳ʱΪԱ¼
-PowerUserPrivilegesRequired=װ˳ʱԹԱ Power User Աݵ¼
-SetupAppRunningError=װ %1 ǰС%n%nرʵȻȷԼȡ˳
-UninstallAppRunningError=жؼ %1 ǰС%n%nرʵȻȷԼȡ˳
-; *** Misc. errors
-ErrorCreatingDir=װĿ¼%1
-ErrorTooManyFilesInDir=Ŀ¼%1дļΪ̫ļ
-; *** Setup common messages
-ExitSetupTitle=˳װ
-ExitSetupMessage=װδɡ˳ᰲװó%n%nʱٴаװɰװ%n%nǷ˳װ?
-AboutSetupMenuItem=ڰװ(&A)...
-AboutSetupTitle=ڰװ
-AboutSetupMessage=%1 汾 %2%n%3%n%n%1 ҳ:%n%4
+SetupLdrStartupMessage=现在将安装 %1。您想要继续吗?
+LdrCannotCreateTemp=不能创建临时文件。安装中断。
+LdrCannotExecTemp=不能执行临时目录中的文件。安装中断。
+HelpTextNote=
+
+; *** 启动错误消息
+LastErrorMessage=%1.%n%n错误 %2: %3
+SetupFileMissing=安装目录中的文件 %1 丢失。请修正这个问题或获取一个新的程序副本。
+SetupFileCorrupt=安装文件已损坏。请获取一个新的程序副本。
+SetupFileCorruptOrWrongVer=安装文件已损坏,或是与这个安装程序的版本不兼容。请修正这个问题或获取新的程序副本。
+InvalidParameter=无效的命令行参数: %n%n%1
+SetupAlreadyRunning=安装程序正在运行。
+WindowsVersionNotSupported=这个程序不支持该版本的计算机运行。
+WindowsServicePackRequired=这个程序要求%1服务包%1或更高。
+NotOnThisPlatform=这个程序将不能运行于 %1。
+OnlyOnThisPlatform=这个程序必须运行于 %1。
+OnlyOnTheseArchitectures=这个程序只能在为下列处理器结构设计的 Windows 版本中进行安装:%n%n%1
+WinVersionTooLowError=这个程序需要 %1 版本 %2 或更高。
+WinVersionTooHighError=这个程序不能安装于 %1 版本 %2 或更高。
+AdminPrivilegesRequired=在安装这个程序时您必须以管理员身份登录。
+PowerUserPrivilegesRequired=在安装这个程序时您必须以管理员身份或有权限的用户组身份登录。
+SetupAppRunningError=安装程序发现 %1 当前正在运行。%n%n请先关闭所有运行的窗口,然后单击“确定”继续,或按“取消”退出。
+UninstallAppRunningError=卸载程序发现 %1 当前正在运行。%n%n请先关闭所有运行的窗口,然后单击“确定”继续,或按“取消”退出。
+
+; *** 启动问题
+PrivilegesRequiredOverrideTitle=选择安装程序模式
+PrivilegesRequiredOverrideInstruction=选择安装模式
+PrivilegesRequiredOverrideText1=%1 可以为所有用户安装(需要管理员权限),或仅为您安装。
+PrivilegesRequiredOverrideText2=%1 只能为您安装,或为所有用户安装(需要管理员权限)。
+PrivilegesRequiredOverrideAllUsers=为所有用户安装(&A)
+PrivilegesRequiredOverrideAllUsersRecommended=为所有用户安装(建议选项)(&A)
+PrivilegesRequiredOverrideCurrentUser=只为我安装(&M)
+PrivilegesRequiredOverrideCurrentUserRecommended=只为我安装(建议选项)(&M)
+
+; *** 其它错误
+ErrorCreatingDir=安装程序不能创建目录“%1”。
+ErrorTooManyFilesInDir=不能在目录“%1”中创建文件,因为里面的文件太多
+
+; *** 安装程序公共消息
+ExitSetupTitle=退出安装程序
+ExitSetupMessage=安装程序未完成安装。如果您现在退出,您的程序将不能安装。%n%n您可以以后再运行安装程序完成安装。%n%n退出安装程序吗?
+AboutSetupMenuItem=关于安装程序(&A)...
+AboutSetupTitle=关于安装程序
+AboutSetupMessage=%1 版本 %2%n%3%n%n%1 主页:%n%4
AboutSetupNote=
TranslatorNote=
-; *** Buttons
-ButtonBack=< һ(&B)
-ButtonNext=һ(&N) >
-ButtonInstall=װ(&I)
-ButtonOK=ȷ
-ButtonCancel=ȡ
-ButtonYes=(&Y)
-ButtonYesToAll=ȫ(&A)
-ButtonNo=(&N)
-ButtonNoToAll=ȫ(&O)
-ButtonFinish=(&F)
-ButtonBrowse=(&B)...
-ButtonWizardBrowse=(&R)...
-ButtonNewFolder=½ļ(&M)
-; *** "Select Language" dialog messages
-SelectLanguageTitle=ѡװ
-SelectLanguageLabel=ѡװʱҪʹõ:
-; *** Common wizard text
-ClickNext=һԼȡ˳װ
+
+; *** 按钮
+ButtonBack=< 上一步(&B)
+ButtonNext=下一步(&N) >
+ButtonInstall=安装(&I)
+ButtonOK=确定
+ButtonCancel=取消
+ButtonYes=是(&Y)
+ButtonYesToAll=全是(&A)
+ButtonNo=否(&N)
+ButtonNoToAll=全否(&O)
+ButtonFinish=完成(&F)
+ButtonBrowse=浏览(&B)...
+ButtonWizardBrowse=浏览(&R)...
+ButtonNewFolder=新建文件夹(&M)
+
+; *** “选择语言”对话框消息
+SelectLanguageTitle=选择安装语言
+SelectLanguageLabel=选择安装时要使用的语言。
+
+; *** 公共向导文字
+ClickNext=单击“下一步”继续,或单击“取消”退出安装程序。
BeveledLabel=
-BrowseDialogTitle=ļ
-BrowseDialogLabel=бѡһļУȻȷ
-NewFolderName=½ļ
-; *** "Welcome" wizard page
-WelcomeLabel1=ӭʹ [name] װ
-WelcomeLabel2=⽫ڼϰװ [name/ver]%n%nرӦóټ
-; *** "Password" wizard page
-WizardPassword=
-PasswordLabel1=˰װ뱣
-PasswordLabel3=ṩ룬ȻһԼִСд
-PasswordEditLabel=(&P):
-IncorrectPassword=벻ȷԡ
-; *** "License Agreement" wizard page
-WizardLicense=Э
-LicenseLabel=ڼǰĶҪϢ
-LicenseLabel3=ĶЭ顣ܴЭſɼװ
-LicenseAccepted=ҽЭ(&A)
-LicenseNotAccepted=ҲЭ(&D)
-; *** "Information" wizard pages
-WizardInfoBefore=Ϣ
-InfoBeforeLabel=ڼǰĶҪϢ
-InfoBeforeClickLabel=üװһ
-WizardInfoAfter=Ϣ
-InfoAfterLabel=ڼǰĶҪϢ
-InfoAfterClickLabel=üװһ
-; *** "User Information" wizard page
-WizardUserInfo=ûϢ
-UserInfoDesc=Ϣ
-UserInfoName=û(&U):
-UserInfoOrg=֯(&O):
-UserInfoSerial=к(&S):
-UserInfoNameRequired=ơ
-; *** "Select Destination Location" wizard page
-WizardSelectDir=ѡĿλ
-SelectDirDesc=Ӧ [name] װ?
-SelectDirLabel3=װὫ [name] װļС
-SelectDirBrowseLabel=ҪһѡļУ
-DiskSpaceMBLabel=Ҫ [mb] MB ô̿ռ䡣
-CannotInstallToNetworkDrive=װװ
-CannotInstallToUNCPath=װװ UNC ·
-InvalidPath=ŵ·(:%n%nC:\APP%n%n)¸ʽ UNC ·:%n%n\\server\share
-InvalidDrive=ѡ UNC ڻɷʡѡ
-DiskSpaceWarningTitle=̿ռ䲻
-DiskSpaceWarning=װҪ %1 KB ÿռװѡ %2 KB ÿռ䡣%n%nǷҪ?
-DirNameTooLong=ļƻ·̫
-InvalidDirName=ļЧ
-BadDirName32=ļܰһַ:%n%n%1
-DirExistsTitle=ļд
-DirExists=ļ:%n%n%1%n%nѴڡǷҪװļ?
-DirDoesntExistTitle=ļв
-DirDoesntExist=ļ:%n%n%1%n%nڡǷҪļ?
-; *** "Select Components" wizard page
-WizardSelectComponents=ѡ
-SelectComponentsDesc=ӦװЩ?
-SelectComponentsLabel2=ѡϣװϣװһԼ
-FullInstallation=ȫװ
+BrowseDialogTitle=浏览文件夹
+BrowseDialogLabel=在下列列表中选择一个文件夹,然后单击“确定”。
+NewFolderName=新建文件夹
+
+; *** “欢迎”向导页
+WelcomeLabel1=欢迎使用 [name] 安装向导
+WelcomeLabel2=现在将安装 [name/ver] 到您的电脑中。%n%n推荐您在继续安装前关闭所有其它应用程序。
+
+; *** “密码”向导页
+WizardPassword=密码
+PasswordLabel1=这个安装程序有密码保护。
+PasswordLabel3=请输入密码,然后单击“下一步”继续。密码区分大小写。
+PasswordEditLabel=密码(&P):
+IncorrectPassword=您输入的密码不正确,请重试。
+
+; *** “许可协议”向导页
+WizardLicense=许可协议
+LicenseLabel=继续安装前请阅读下列重要信息。
+LicenseLabel3=请仔细阅读下列许可协议。您在继续安装前必须同意这些协议条款。
+LicenseAccepted=我同意此协议(&A)
+LicenseNotAccepted=我不同意此协议(&D)
+
+; *** “信息”向导页
+WizardInfoBefore=信息
+InfoBeforeLabel=请在继续安装前阅读下列重要信息。
+InfoBeforeClickLabel=如果您想继续安装,单击“下一步”。
+WizardInfoAfter=信息
+InfoAfterLabel=请在继续安装前阅读下列重要信息。
+InfoAfterClickLabel=如果您想继续安装,单击“下一步”。
+
+; *** “用户信息”向导页
+WizardUserInfo=用户信息
+UserInfoDesc=请输入您的信息。
+UserInfoName=用户名(&U):
+UserInfoOrg=组织(&O):
+UserInfoSerial=序列号(&S):
+UserInfoNameRequired=您必须输入名字。
+
+; *** “选择目标目录”向导面
+WizardSelectDir=选择目标位置
+SelectDirDesc=您想将 [name] 安装在什么地方?
+SelectDirLabel3=安装程序将安装 [name] 到下列文件夹中。
+SelectDirBrowseLabel=单击“下一步”继续。如果您想选择其它文件夹,单击“浏览”。
+DiskSpaceGBLabel=至少需要有 [gb] GB 的可用磁盘空间。
+DiskSpaceMBLabel=至少需要有 [mb] MB 的可用磁盘空间。
+CannotInstallToNetworkDrive=安装程序无法安装到一个网络驱动器。
+CannotInstallToUNCPath=安装程序无法安装到一个UNC路径。
+InvalidPath=您必须输入一个带驱动器卷标的完整路径,例如:%n%nC:\APP%n%n或下列形式的 UNC 路径:%n%n\\server\share
+InvalidDrive=您选定的驱动器或 UNC 共享不存在或不能访问。请选选择其它位置。
+DiskSpaceWarningTitle=没有足够的磁盘空间
+DiskSpaceWarning=安装程序至少需要 %1 KB 的可用空间才能安装,但选定驱动器只有 %2 KB 的可用空间。%n%n您一定要继续吗?
+DirNameTooLong=文件夹名或路径太长。
+InvalidDirName=文件夹名是无效的。
+BadDirName32=文件夹名不能包含下列任何字符:%n%n%1
+DirExistsTitle=文件夹存在
+DirExists=文件夹:%n%n%1%n%n已经存在。您一定要安装到这个文件夹中吗?
+DirDoesntExistTitle=文件夹不存在
+DirDoesntExist=文件夹:%n%n%1%n%n不存在。您想要创建此目录吗?
+
+; *** “选择组件”向导页
+WizardSelectComponents=选择组件
+SelectComponentsDesc=您想安装哪些程序的组件?
+SelectComponentsLabel2=选择您想要安装的组件;清除您不想安装的组件。然后单击“下一步”继续。
+FullInstallation=完全安装
; if possible don't translate 'Compact' as 'Minimal' (I mean 'Minimal' in your language)
-CompactInstallation=లװ
-CustomInstallation=Զ尲װ
-NoUninstallWarningTitle=
-NoUninstallWarning=װѰװ:%n%n%1%n%nȡѡЩжǡ%n%nǷҪ?
+CompactInstallation=简洁安装
+CustomInstallation=自定义安装
+NoUninstallWarningTitle=组件存在
+NoUninstallWarning=安装程序侦测到下列组件已在您的电脑中安装。:%n%n%1%n%n取消选定这些组件将不能卸载它们。%n%n您一定要继续吗?
ComponentSize1=%1 KB
ComponentSize2=%1 MB
-ComponentsDiskSpaceMBLabel=ǰѡҪ [mb] MB ̿ռ䡣
-; *** "Select Additional Tasks" wizard page
-WizardSelectTasks=ѡ
-SelectTasksDesc=ӦִЩ?
-SelectTasksLabel2=ѡװ [name] ʱϣװִеȻһ
-; *** "Select Start Menu Folder" wizard page
-WizardSelectProgramGroup=ѡʼ˵ļ
-SelectStartMenuFolderDesc=װӦĿݷʽõ?
-SelectStartMenuFolderLabel3=װ¿ʼ˵ļдóĿݷʽ
-SelectStartMenuFolderBrowseLabel=ҪһѡļУ
-MustEnterGroupName=ļ
-GroupNameTooLong=ļƻ·̫
-InvalidGroupName=ļЧ
-BadGroupName=ļܱһַ:%n%n%1
-NoProgramGroupCheck2=ʼ˵ļ(&D)
-; *** "Ready to Install" wizard page
-WizardReady=װ
-ReadyLabel1=װڼϰװ [name]
-ReadyLabel2a=װԼװ鿴κ""
-ReadyLabel2b=װԼװ
-ReadyMemoUserInfo=ûϢ:
-ReadyMemoDir=Ŀλ:
-ReadyMemoType=װ:
-ReadyMemoComponents=ѡ:
-ReadyMemoGroup=ʼ˵ļ:
-ReadyMemoTasks=:
-; *** "Preparing to Install" wizard page
-WizardPreparing=װ
-PreparingDesc=װڼϰװ [name]
-PreviousInstallNotCompleted=һİװ/ɾδɡɸðװ%n%nаװ [name] İװ
-CannotContinue=װ뵥"ȡ"˳
-ApplicationsFound=ӦóʹҪͨװиµļװԶرЩӦó
-ApplicationsFound2=ӦóʹҪͨװиµļװԶرЩӦóɰװװӦó
-CloseApplications=ԶرӦó(&A)
-DontCloseApplications=رӦó(&D)
-ErrorCloseApplications=װԶرӦóڼ֮ǰȹرʹͨװиµļӦó
-; *** "Installing" wizard page
-WizardInstalling=ڰװ
-InstallingLabel=װڼϰװ [name]Եȡ
-; *** "Setup Completed" wizard page
-FinishedHeadingLabel= [name] װ
-FinishedLabelNoIcons=װڼɰװ [name]
-FinishedLabel=װڼɰװ [name]ͨѡװĿݷʽӦó
-ClickFinish=ɡ˳װ
-FinishedRestartLabel=Ҫ [name] İװװǷҪ?
-FinishedRestartMessage=Ҫ [name] İװװ%n%nǷҪ?
-ShowReadmeCheck=ǣϣ鿴 README ļ
-YesRadio=ǣ(&Y)
-NoRadio=ҽԺ(&N)
-; used for example as 'Run MyProg.exe'
-RunEntryExec= %1
-; used for example as 'View Readme.txt'
-RunEntryShellExec=鿴 %1
-; *** "Setup Needs the Next Disk" stuff
-ChangeDiskTitle=װҪһ
-SelectDiskLabel2= %1 ȷ%n%n˴ϵļļļҵȷ·
-PathLabel=·(&P):
-FileNotInDir2=ڡ%2λļ%1ȷĴ̻ѡļС
-SelectDirectoryLabel=ָһ̵λá
-; *** Installation phase messages
-SetupAborted=װδɡ%n%nⲢаװ
-EntryAbortRetryIgnore=ԡٴγԣԡԼֹȡװ
-; *** Installation status messages
-StatusClosingApplications=ڹرӦó...
-StatusCreateDirs=ڴĿ¼...
-StatusExtractFiles=ڽѹļ...
-StatusCreateIcons=ڴݷʽ...
-StatusCreateIniEntries=ڴ INI ...
-StatusCreateRegistryEntries=ڴע...
-StatusRegisterFiles=עļ...
-StatusSavingUninstall=ڱжϢ...
-StatusRunProgram=ɰװ...
-StatusRestartingApplications=Ӧó...
-StatusRollback=ڻ˸...
-; *** Misc. errors
-ErrorInternal2=ڲ: %1
-ErrorFunctionFailedNoCode=%1 ʧ
-ErrorFunctionFailed=%1 ʧܣ %2
-ErrorFunctionFailedWithMessage=%1 ʧܣ %2%n%3
-ErrorExecutingProgram=ִļ:%n%1
-; *** Registry errors
-ErrorRegOpenKey=עʱ:%n%1\%2
-ErrorRegCreateKey=עʱ:%n%1\%2
-ErrorRegWriteKey=дעʱ:%n%1\%2
-; *** INI errors
-ErrorIniEntry=ļ%1д INI ʱ
-; *** File copying errors
-FileAbortRetryIgnore=ԡٴβԡļ(˲)ֹȡװ
-FileAbortRetryIgnore2=ԡٴβԡԼ(˲)ֹȡװ
-SourceIsCorrupted=Դļ
-SourceDoesntExist=Դļ%1
-ExistingFileReadOnly=ļΪֻ״̬%n%nԡɾֻԲԣԡļֹȡװ
-ErrorReadingExistingDest=Զȡļʱ:
-FileExists=ļѴڡ%n%nǷҪװ?
-ExistingFileNewer=ļȰװװļ¡鱣ļ%n%nǷҪļ?
-ErrorChangingAttr=ԸļԳ:
-ErrorCreatingTemp=ĿĿ¼ļʱ:
-ErrorReadingSource=ԶȡԴļʱ:
-ErrorCopying=Ըļʱ:
-ErrorReplacingExistingFile=滻ļʱ:
-ErrorRestartReplace=RestartReplace ʧ:
-ErrorRenamingTemp=ĿĿ¼ļʱ:
-ErrorRegisterServer=ע DLL/OCX: %1
-ErrorRegSvr32Failed=RegSvr32 ʧܣ˳Ϊ %1
-ErrorRegisterTypeLib=עͿ: %1
-; *** Post-installation errors
-ErrorOpeningReadme=Դ README ļʱ
-ErrorRestartingComputer=װִֶд˲
-; *** Uninstaller messages
-UninstallNotFound=ļ%1ڡװ
-UninstallOpenError=ļ%1ж
-UninstallUnsupportedVer=ж־%1ĸʽ˰汾жسʶж
-UninstallUnknownEntry=ж־зδ֪Ŀ(%1)
-ConfirmUninstall=ȷҪɾ %1 ͼȫ?
-UninstallOnlyOnWin64= 64 λ Windows жش˰װ
-OnlyAdminCanUninstall=йȨûſжش˰װ
-UninstallStatusLabel=Ӽɾ %1Եȡ
-UninstalledAll=ѳɹӼɾ %1
-UninstalledMost=%1 жɡ%n%nɾһЩԪءɽֶɾ
-UninstalledAndNeedsRestart=Ҫ %1 жأ%n%nǷҪ?
-UninstallDataCorrupted=%1ļж
-; *** Uninstallation phase messages
-ConfirmDeleteSharedFileTitle=ɾļ?
-ConfirmDeleteSharedFile2=ϵͳʾ¹ļٱκγʹáǷҪжɾ˹ļ?%n%nгʹôļɾܲСȷѡļסϵͳϲκ⡣
-SharedFileNameLabel=ļ:
-SharedFileLocationLabel=λ:
-WizardUninstalling=ж״̬
-StatusUninstalling=ж %1...
+ComponentsDiskSpaceGBLabel=当前选择的组件至少需要 [gb] GB 的磁盘空间。
+ComponentsDiskSpaceMBLabel=当前选择的组件至少需要 [mb] MB 的磁盘空间。
+
+; *** “选择附加任务”向导页
+WizardSelectTasks=选择附加任务
+SelectTasksDesc=您想要安装程序执行哪些附加任务?
+SelectTasksLabel2=选择您想要安装程序在安装 [name] 时执行的附加任务,然后单击“下一步”。
+
+; *** “选择开始菜单文件夹”向导页
+WizardSelectProgramGroup=选择开始菜单文件夹
+SelectStartMenuFolderDesc=您想在哪里放置程序的快捷方式?
+SelectStartMenuFolderLabel3=安装程序现在将在下列开始菜单文件夹中创建程序的快捷方式。
+SelectStartMenuFolderBrowseLabel=单击“下一步”继续。如果您想选择其它文件夹,单击“浏览”。
+MustEnterGroupName=您必须输入一个文件夹名。
+GroupNameTooLong=文件夹名或路径太长。
+InvalidGroupName=文件夹名是无效的。
+BadGroupName=文件夹名不能包含下列任何字符:%n%n%1
+NoProgramGroupCheck2=不创建开始菜单文件夹(&D)
+
+; *** “准备安装”向导页
+WizardReady=准备安装
+ReadyLabel1=安装程序现在准备开始安装 [name] 到您的电脑中。
+ReadyLabel2a=单击“安装”继续此安装程序。如果您想要回顾或改变设置,请单击“上一步”。
+ReadyLabel2b=单击“安装”继续此安装程序?
+ReadyMemoUserInfo=用户信息:
+ReadyMemoDir=目标位置:
+ReadyMemoType=安装类型:
+ReadyMemoComponents=选定组件:
+ReadyMemoGroup=开始菜单文件夹:
+ReadyMemoTasks=附加任务:
+
+; *** “正在准备安装”向导页
+WizardPreparing=正在准备安装
+PreparingDesc=安装程序正在准备安装 [name] 到您的电脑中。
+PreviousInstallNotCompleted=先前程序的安装/卸载未完成。您需要重新启动您的电脑才能完成安装。%n%n在重新启动电脑后,再运行安装完成 [name] 的安装。
+CannotContinue=安装程序不能继续。请单击“取消”退出。
+ApplicationsFound=下列应用程序正在使用的文件需要更新设置。它是建议您允许安装程序自动关闭这些应用程序。
+ApplicationsFound2=下列应用程序正在使用的文件需要更新设置。它是建议您允许安装程序自动关闭这些应用程序。安装完成后,安装程序将尝试重新启动应用程序。
+CloseApplications=自动关闭该应用程序(&A)
+DontCloseApplications=不要关闭该应用程序(D)
+ErrorCloseApplications=安装程序无法自动关闭所有应用程序。在继续之前,我们建议您关闭所有使用需要更新的安装程序文件。
+PrepareToInstallNeedsRestart=安装程序必须重新启动计算机。重新启动计算机后,请再次运行安装程序以完成 [name] 的安装。%n%n是否立即重新启动?
+
+; *** “正在安装”向导页
+WizardInstalling=正在安装
+InstallingLabel=安装程序正在安装 [name] 到您的电脑中,请稍等。
+
+; *** “安装完成”向导页
+FinishedHeadingLabel=[name] 安装完成
+FinishedLabelNoIcons=安装程序已在您的电脑中安装了 [name]。
+FinishedLabel=安装程序已在您的电脑中安装了 [name]。此应用程序可以通过选择安装的快捷方式运行。
+ClickFinish=单击“完成”退出安装程序。
+FinishedRestartLabel=要完成 [name] 的安装,安装程序必须重新启动您的电脑。您想现在重新启动吗?
+FinishedRestartMessage=要完成 [name] 的安装,安装程序必须重新启动您的电脑。%n%n您想现在重新启动吗?
+ShowReadmeCheck=是,您想查阅自述文件
+YesRadio=是,立即重新启动电脑(&Y)
+NoRadio=否,稍后重新启动电脑(&N)
+; 用于象“运行 MyProg.exe”
+RunEntryExec=运行 %1
+; 用于象“查阅 Readme.txt”
+RunEntryShellExec=查阅 %1
+
+; *** “安装程序需要下一张磁盘”提示
+ChangeDiskTitle=安装程序需要下一张磁盘
+SelectDiskLabel2=请插入磁盘 %1 并单击“确定”。%n%n如果这个磁盘中的文件不能在不同于下列显示的文件夹中找到,输入正确的路径或单击“浏览”。
+PathLabel=路径(&P):
+FileNotInDir2=文件“%1”不能在“%2”定位。请插入正确的磁盘或选择其它文件夹。
+SelectDirectoryLabel=请指定下一张磁盘的位置。
+
+; *** 安装状态消息
+SetupAborted=安装程序未完成安装。%n%n请修正这个问题并重新运行安装程序。
+AbortRetryIgnoreSelectAction=选项
+AbortRetryIgnoreRetry=重试(&T)
+AbortRetryIgnoreIgnore=忽略错误并继续(&I)
+AbortRetryIgnoreCancel=关闭安装程序
+
+; *** 安装状态消息
+StatusClosingApplications=正在关闭应用程序...
+StatusCreateDirs=正在创建目录...
+StatusExtractFiles=正在解压缩文件...
+StatusCreateIcons=正在创建快捷方式...
+StatusCreateIniEntries=正在创建 INI 条目...
+StatusCreateRegistryEntries=正在创建注册表条目...
+StatusRegisterFiles=正在注册文件...
+StatusSavingUninstall=正在保存卸载信息...
+StatusRunProgram=正在完成安装...
+StatusRestartingApplications=正在重启应用程序...
+StatusRollback=正在撤销更改...
+
+; *** 其它错误
+ErrorInternal2=内部错误: %1
+ErrorFunctionFailedNoCode=%1 失败
+ErrorFunctionFailed=%1 失败;错误代码 %2
+ErrorFunctionFailedWithMessage=%1 失败;错误代码 %2.%n%3
+ErrorExecutingProgram=不能执行文件:%n%1
+
+; *** 注册表错误
+ErrorRegOpenKey=打开注册表项时出错:%n%1\%2
+ErrorRegCreateKey=创建注册表项时出错:%n%1\%2
+ErrorRegWriteKey=写入注册表项时出错:%n%1\%2
+
+; *** INI 错误
+ErrorIniEntry=在文件“%1”创建 INI 项目错误。
+
+; *** 文件复制错误
+FileAbortRetryIgnoreSkipNotRecommended=跳过这个文件 (不推荐)(&S)
+FileAbortRetryIgnoreIgnoreNotRecommended=忽略错误并继续 (不推荐)(&I)
+SourceIsCorrupted=源文件已损坏
+SourceDoesntExist=源文件“%1”不存在
+ExistingFileReadOnly2=无法替换现有文件,因为它是只读的。
+ExistingFileReadOnlyRetry=移除只读属性并重试(&R)
+ExistingFileReadOnlyKeepExisting=保留现有文件(&K)
+ErrorReadingExistingDest=尝试读取现有文件时发生一个错误:
+FileExists=文件已经存在。%n%n您想要安装程序覆盖它吗?
+ExistingFileNewer=现有的文件新与安装程序要安装的文件。推荐您保留现有文件。%n%n您想要保留现有的文件吗?
+ErrorChangingAttr=尝试改变下列现有的文件的属性时发生一个错误:
+ErrorCreatingTemp=尝试在目标目录创建文件时发生一个错误:
+ErrorReadingSource=尝试读取下列源文件时发生一个错误:
+ErrorCopying=尝试复制下列文件时发生一个错误:
+ErrorReplacingExistingFile=尝试替换现有的文件时发生错误:
+ErrorRestartReplace=重启电脑后替换文件失败:
+ErrorRenamingTemp=尝试重新命名以下目标目录中的一个文件时发生错误:
+ErrorRegisterServer=不能注册 DLL/OCX: %1
+ErrorRegSvr32Failed=RegSvr32 失败;退出代码 %1
+ErrorRegisterTypeLib=不能注册类型库: %1
+
+; *** 卸载显示名字标记
+; used for example as 'My Program (32-bit)'
+UninstallDisplayNameMark=%1 (%2)
+; used for example as 'My Program (32-bit, All users)'
+UninstallDisplayNameMarks=%1 (%2, %3)
+UninstallDisplayNameMark32Bit=32位
+UninstallDisplayNameMark64Bit=64位
+UninstallDisplayNameMarkAllUsers=所有用户
+UninstallDisplayNameMarkCurrentUser=当前用户
+
+; *** 安装后错误
+ErrorOpeningReadme=当尝试打开自述文件时发生一个错误。
+ErrorRestartingComputer=安装程序不能重新启动电脑,请手动重启。
+
+; *** 卸载消息
+UninstallNotFound=文件“%1”不存在。不能卸载。
+UninstallOpenError=文件“%1”不能打开。不能卸载。
+UninstallUnsupportedVer=卸载日志文件“%1”有未被这个版本的卸载器承认的格式。不能卸载
+UninstallUnknownEntry=在卸载日志中遇到一个未知的条目 (%1)
+ConfirmUninstall=您确认想要完全删除 %1 及它的所有组件吗?
+UninstallOnlyOnWin64=这个安装程序只能在 64 位 Windows 中进行卸载。
+OnlyAdminCanUninstall=这个安装的程序只能是有管理员权限的用户才能卸载。
+UninstallStatusLabel=正在从您的电脑中删除 %1,请等待。
+UninstalledAll=%1 已顺利地从您的电脑中删除。
+UninstalledMost=%1 卸载完成。%n%n有一些内容不能被删除。您可以手工删除它们。
+UninstalledAndNeedsRestart=要完成 %1 的卸载,您的电脑必须重新启动。%n%n您现在想重新启动电脑吗?
+UninstallDataCorrupted=“%1”文件被破坏,不能卸载
+
+; *** 卸载状态消息
+ConfirmDeleteSharedFileTitle=删除共享文件吗?
+ConfirmDeleteSharedFile2=系统中包含的下列共享文件已经不被其它程序使用。您想要卸载程序删除这些共享文件吗?%n%n如果这些文件被删除,但还有程序正在使用这些文件,这些程序可能不能正确执行。如果您不能确定,选择“否”。把这些文件保留在系统中以免引起问题。
+SharedFileNameLabel=文件名:
+SharedFileLocationLabel=位置:
+WizardUninstalling=卸载状态
+StatusUninstalling=正在卸载 %1...
+
; *** Shutdown block reasons
-ShutdownBlockReasonInstallingApp=ڰװ %1
-ShutdownBlockReasonUninstallingApp=ж %1
+ShutdownBlockReasonInstallingApp=正在安装 %1.
+ShutdownBlockReasonUninstallingApp=正在卸载 %1.
+
; The custom messages below aren't used by Setup itself, but if you make
; use of them in your scripts, you'll want to translate them.
+
[CustomMessages]
-NameAndVersion=%1 汾 %2
-AdditionalIcons=ݷʽ:
-CreateDesktopIcon=ݷʽ(&D)
-CreateQuickLaunchIcon=ݷʽ(&Q)
-ProgramOnTheWeb=Web ϵ %1
-UninstallProgram=ж %1
-LaunchProgram= %1
-AssocFileExtension= %1 %2 ļչ(&A)
-AssocingFileExtension= %1 %2 ļչ...
-AutoStartProgramGroupDescription=:
-AutoStartProgram=Զ %1
-AddonHostProgramNotFound=ѡļжλ %1%n%nǷҪ?
\ No newline at end of file
+
+NameAndVersion=%1 版本 %2
+AdditionalIcons=附加快捷方式:
+CreateDesktopIcon=创建桌面快捷方式(&D)
+CreateQuickLaunchIcon=创建快速运行栏快捷方式(&Q)
+ProgramOnTheWeb=%1 网站
+UninstallProgram=卸载 %1
+LaunchProgram=运行 %1
+AssocFileExtension=将 %2 文件扩展名与 %1 建立关联(&A)
+AssocingFileExtension=正在将 %2 文件扩展名与 %1 建立关联...
+AutoStartProgramGroupDescription=启动组:
+AutoStartProgram=自动启动 %1
+AddonHostProgramNotFound=%1无法找到您所选择的文件夹。%n%n您想要继续吗?
+
diff --git a/build/win32/i18n/Default.zh-tw.isl b/build/win32/i18n/Default.zh-tw.isl
index 4746349e191bd..fb748761f99e2 100644
--- a/build/win32/i18n/Default.zh-tw.isl
+++ b/build/win32/i18n/Default.zh-tw.isl
@@ -1,298 +1,359 @@
-; *** Inno Setup version 5.5.3+ Traditional Chinese messages ***
+; *** Inno Setup version 6.0.0+ Chinese Traditional messages ***
;
-; To download user-contributed translations of this file, go to:
-; http://www.jrsoftware.org/files/istrans/
+; Name: John Wu, mr.johnwu@gmail.com
+; Base on 5.5.3+ translations by Samuel Lee, Email: 751555749@qq.com
+; Translation based on network resource
;
-; Note: When translating this text, do not add periods (.) to the end of
-; messages that didn't have them already, because on those messages Inno
-; Setup adds the periods automatically (appending a period would result in
-; two periods being displayed).
+
[LangOptions]
; The following three entries are very important. Be sure to read and
; understand the '[LangOptions] section' topic in the help file.
-LanguageName=Traditional Chinese
+; If Language Name display incorrect, uncomment next line
+LanguageName=<7e41><9ad4><4e2d><6587>
LanguageID=$0404
-LanguageCodePage=950
+LanguageCodepage=950
; If the language you are translating to requires special font faces or
; sizes, uncomment any of the following entries and change them accordingly.
-;DialogFontName=
-;DialogFontSize=8
-;WelcomeFontName=Verdana
-;WelcomeFontSize=12
-;TitleFontName=Arial
-;TitleFontSize=29
-;CopyrightFontName=Arial
-;CopyrightFontSize=8
+DialogFontName=新細明體
+DialogFontSize=9
+TitleFontName=Arial
+TitleFontSize=28
+WelcomeFontName=新細明體
+WelcomeFontSize=12
+CopyrightFontName=新細明體
+CopyrightFontSize=9
+
[Messages]
+
; *** Application titles
-SetupAppTitle=w˵{
-SetupWindowTitle=w˵{ - %1
-UninstallAppTitle=Ѱw
-UninstallAppFullTitle=%1 Ѱw
+SetupAppTitle=安裝程式
+SetupWindowTitle=%1 安裝程式
+UninstallAppTitle=解除安裝
+UninstallAppFullTitle=解除安裝 %1
+
; *** Misc. common
-InformationTitle=T
-ConfirmTitle=T{
-ErrorTitle=~
+InformationTitle=訊息
+ConfirmTitle=確認
+ErrorTitle=錯誤
+
; *** SetupLdr messages
-SetupLdrStartupMessage=o|w %1Cn~?
-LdrCannotCreateTemp=LkإȦsɡCwˤw
-LdrCannotExecTemp=LkȦsؿɮסCwˤw
+SetupLdrStartupMessage=這將會安裝 %1。您想要繼續嗎?
+LdrCannotCreateTemp=無法建立暫存檔案。安裝程式將會結束。
+LdrCannotExecTemp=無法執行暫存檔案。安裝程式將會結束。
+HelpTextNote=
+
; *** Startup error messages
-LastErrorMessage=%1C%n%n~ %2: %3
-SetupFileMissing=w˥ؿʤɮ %1CЭץDAέso{sƥC
-SetupFileCorrupt=w˵{ɮפwlCЭsoӵ{ƥC
-SetupFileCorruptOrWrongVer=w˵{ɮפwlAΤۮePw˵{CЭץDAέso{sƥC
-InvalidParameter=bROCWǻFLĪѼ:%n%n%1
-SetupAlreadyRunning=w˵{wb椤C
-WindowsVersionNotSupported={䴩qҰ檺 Windows C
-WindowsServicePackRequired={ݭn %1 Service Pack %2 ΧsC
-NotOnThisPlatform={|b %1 WC
-OnlyOnThisPlatform={b %1 WC
-OnlyOnTheseArchitectures={uiw˦bMUCBz[c]p Windows W:%n%n%1
-MissingWOW64APIs=z檺 Windows tw˵{ 64 줸w˩һݪ\CYnץDAЦw Service Pack %1C
-WinVersionTooLowError={ݭn %1 %2 ΧsC
-WinVersionTooHighError={Lkw˦b %1 %2 ΧsWC
-AdminPrivilegesRequired=w˦{ɡAHtκznJC
-PowerUserPrivilegesRequired=zw˦{ɡAHtκz Power Users sժnJC
-SetupAppRunningError=wˮɰ %1 ثeb椤C%n%nХߧYҦCYn~AЫ@U [Tw]; YnAЫ@U []C
-UninstallAppRunningError=Ѱwˮɰ %1 ثeb椤C%n%nХߧYҦCYn~AЫ@U [Tw]; YnAЫ@U []C
+LastErrorMessage=%1%n%n錯誤 %2: %3
+SetupFileMissing=安裝資料夾中遺失檔案 %1。請修正此問題或重新取得此軟體。
+SetupFileCorrupt=安裝檔案已經損毀。請重新取得此軟體。
+SetupFileCorruptOrWrongVer=安裝檔案已經損毀,或與安裝程式的版本不符。請重新取得此軟體。
+InvalidParameter=某個無效的變量已被傳遞到了命令列:%n%n%1
+SetupAlreadyRunning=安裝程式已經在執行。
+WindowsVersionNotSupported=本安裝程式並不支援目前在電腦所運行的 Windows 版本。
+WindowsServicePackRequired=本安裝程式需要 %1 Service Pack %2 或更新。
+NotOnThisPlatform=這個程式無法在 %1 執行。
+OnlyOnThisPlatform=這個程式必須在 %1 執行。
+OnlyOnTheseArchitectures=這個程式只能在專門為以下處理器架構而設計的 Windows 上安裝:%n%n%1
+WinVersionTooLowError=這個程式必須在 %1 版本 %2 或以上的系統執行。
+WinVersionTooHighError=這個程式無法安裝在 %1 版本 %2 或以上的系統。
+AdminPrivilegesRequired=您必須登入成系統管理員以安裝這個程式。
+PowerUserPrivilegesRequired=您必須登入成具有系統管理員或 Power User 權限的使用者以安裝這個程式。
+SetupAppRunningError=安裝程式偵測到 %1 正在執行。%n%n請關閉該程式後按 [確定] 繼續,或按 [取消] 離開。
+UninstallAppRunningError=解除安裝程式偵測到 %1 正在執行。%n%n請關閉該程式後按 [確定] 繼續,或按 [取消] 離開。
+
+; *** Startup questions
+PrivilegesRequiredOverrideTitle=選擇安裝程式安裝模式
+PrivilegesRequiredOverrideInstruction=選擇安裝模式
+PrivilegesRequiredOverrideText1=可以為所有使用者安裝 %1 (需要系統管理權限),或是僅為您安裝。
+PrivilegesRequiredOverrideText2=可以僅為您安裝 %1,或是為所有使用者安裝 (需要系統管理權限)。
+PrivilegesRequiredOverrideAllUsers=為所有使用者安裝 (&A)
+PrivilegesRequiredOverrideAllUsersRecommended=為所有使用者安裝 (建議選項) (&A)
+PrivilegesRequiredOverrideCurrentUser=僅為我安裝 (&M)
+PrivilegesRequiredOverrideCurrentUserRecommended=僅為我安裝 (建議選項) (&M)
+
; *** Misc. errors
-ErrorCreatingDir=w˵{Lkإߥؿ "%1"
-ErrorTooManyFilesInDir=]ؿ "%1" ]tӦhɮסAҥHLkb䤤إɮ
+ErrorCreatingDir=安裝程式無法建立資料夾“%1”。
+ErrorTooManyFilesInDir=無法在資料夾“%1”內建立檔案,因為資料夾內有太多的檔案。
+
; *** Setup common messages
-ExitSetupTitle=w
-ExitSetupMessage=w˥CYߧYAN|w˵{C%n%nziHyAw˵{ӧwˡC%n%nnw˶?
-AboutSetupMenuItem=w˵{(&A)...
-AboutSetupTitle=w˵{
-AboutSetupMessage=%1 %2%n%3%n%n%1 :%n%4
+ExitSetupTitle=結束安裝程式
+ExitSetupMessage=安裝尚未完成。如果您現在結束安裝程式,這個程式將不會被安裝。%n%n您可以稍後再執行安裝程式以完成安裝程序。您現在要結束安裝程式嗎?
+AboutSetupMenuItem=關於安裝程式(&A)...
+AboutSetupTitle=關於安裝程式
+AboutSetupMessage=%1 版本 %2%n%3%n%n%1 網址:%n%4
AboutSetupNote=
TranslatorNote=
+
; *** Buttons
-ButtonBack=< W@B(&B)
-ButtonNext=U@B(&N) >
-ButtonInstall=w(&I)
-ButtonOK=Tw
-ButtonCancel=
-ButtonYes=O(&Y)
-ButtonYesToAll=ҬO(&A)
-ButtonNo=_(&N)
-ButtonNoToAll=ҧ_(&O)
-ButtonFinish=(&F)
-ButtonBrowse=s(&B)...
-ButtonWizardBrowse=s(&R)...
-ButtonNewFolder=إ߷sƧ(&M)
+ButtonBack=< 上一步(&B)
+ButtonInstall=安裝(&I)
+ButtonNext=下一步(&N) >
+ButtonOK=確定
+ButtonCancel=取消
+ButtonYes=是(&Y)
+ButtonYesToAll=全部皆是(&A)
+ButtonNo=否(&N)
+ButtonNoToAll=全部皆否(&O)
+ButtonFinish=完成(&F)
+ButtonBrowse=瀏覽(&B)...
+ButtonWizardBrowse=瀏覽(&R)...
+ButtonNewFolder=建立新資料夾(&M)
+
; *** "Select Language" dialog messages
-SelectLanguageTitle=w˵{y
-SelectLanguageLabel=w˴ҭnϥΪy:
+SelectLanguageTitle=選擇安裝語言
+SelectLanguageLabel=選擇在安裝過程中使用的語言:
+
; *** Common wizard text
-ClickNext=Yn~AЫ@U [U@B]; YnwˡAЫ@U []C
+ClickNext=按 [下一步] 繼續安裝,或按 [取消] 結束安裝程式。
BeveledLabel=
-BrowseDialogTitle=sƧ
-BrowseDialogLabel=бqUCM椤ƧAM@U [Tw]C
-NewFolderName=sWƧ
+BrowseDialogTitle=瀏覽資料夾
+BrowseDialogLabel=在下面的資料夾列表中選擇一個資料夾,然後按 [確定]。
+NewFolderName=新資料夾
+
; *** "Welcome" wizard page
-WelcomeLabel1=wϥ [name] w˺F
-WelcomeLabel2=o|bzqWw [name/ver]C%n%nijzҦLε{AMA~C
+WelcomeLabel1=歡迎使用 [name] 安裝程式
+WelcomeLabel2=這個安裝程式將會安裝 [name/ver] 到您的電腦。%n%n我們強烈建議您在安裝過程中關閉其它的應用程式,以避免與安裝程式發生沖突。
+
; *** "Password" wizard page
-WizardPassword=KX
-PasswordLabel1=w˨KXO@C
-PasswordLabel3=дѱKXAM@U [U@B] H~CKXϤjpgC
-PasswordEditLabel=KX(&P):
-IncorrectPassword=JKXTCЦAդ@C
+WizardPassword=密碼
+PasswordLabel1=這個安裝程式具有密碼保護。
+PasswordLabel3=請輸入密碼,然後按 [下一步] 繼續。密碼是區分大小寫的。
+PasswordEditLabel=密碼(&P):
+IncorrectPassword=您輸入的密碼不正確,請重新輸入。
+
; *** "License Agreement" wizard page
-WizardLicense=vX
-LicenseLabel=Х\ŪUCnTA~C
-LicenseLabel3=о\ŪUCvXCzXڡA~~wˡC
-LicenseAccepted=ڱX(&A)
-LicenseNotAccepted=ڤX(&D)
+WizardLicense=授權合約
+LicenseLabel=請閱讀以下授權合約。
+LicenseLabel3=請閱讀以下授權合約,您必須接受合約的各項條款才能繼續安裝。
+LicenseAccepted=我同意(&A)
+LicenseNotAccepted=我不同意(&D)
+
; *** "Information" wizard pages
-WizardInfoBefore=T
-InfoBeforeLabel=Х\ŪUCnTA~C
-InfoBeforeClickLabel=zdzƦnn~wˮɡAЫ@U [U@B]C
-WizardInfoAfter=T
-InfoAfterLabel=Х\ŪUCnTA~C
-InfoAfterClickLabel=zdzƦnn~wˮɡAЫ@U [U@B]C
+WizardInfoBefore=訊息
+InfoBeforeLabel=在繼續安裝之前請閱讀以下重要資訊。
+InfoBeforeClickLabel=當您準備好繼續安裝,請按 [下一步]。
+WizardInfoAfter=訊息
+InfoAfterLabel=在繼續安裝之前請閱讀以下重要資訊。
+InfoAfterClickLabel=當您準備好繼續安裝,請按 [下一步]。
+
; *** "User Information" wizard page
-WizardUserInfo=ϥΪ̸T
-UserInfoDesc=пJzTC
-UserInfoName=ϥΪ̦W(&U):
-UserInfoOrg=´(&O):
-UserInfoSerial=Ǹ(&S):
-UserInfoNameRequired=JW١C
+WizardUserInfo=使用者資訊
+UserInfoDesc=請輸入您的資料。
+UserInfoName=使用者名稱(&U):
+UserInfoOrg=組織(&O):
+UserInfoSerial=序號(&S):
+UserInfoNameRequired=您必須輸入您的名稱。
+
; *** "Select Destination Location" wizard page
-WizardSelectDir=تam
-SelectDirDesc=N [name] w˦bB?
-SelectDirLabel3=w˵{|N [name] w˦bUCƧC
-SelectDirBrowseLabel=Yn~AЫ@U [U@B]CYzQPƧAЫ@U [s]C
-DiskSpaceMBLabel=ܤֶ [mb] MB iκϺЪŶC
-CannotInstallToNetworkDrive=w˵{Lkw˨ϺоC
-CannotInstallToUNCPath=w˵{Lkw˨ UNC |C
-InvalidPath=J]tϺоN|AҦp:%n%nC:\APP%n%nοJUC榡 UNC |:%n%n\\A\@
-InvalidDrive=Ϻо UNC @ΤsbεLksCпLϺо UNC @ΡC
-DiskSpaceWarningTitle=ϺЪŶ
-DiskSpaceWarning=w˵{ܤֻݭn %1 KB iΪŶ~wˡAҿϺоiΪŶu %2 KBC%n%nn~?
-DirNameTooLong=ƧW٩θ|LC
-InvalidDirName=ƧWٵLġC
-BadDirName32=ƧW٤o]tUC@r:%n%n%1
-DirExistsTitle=Ƨwsb
-DirExists=wƧ %n%n%1%n%nCnw˨ӸƧ?
-DirDoesntExistTitle=Ƨsb
-DirDoesntExist=Ƨ %n%n%1%n%n sbCnإ߸ӸƧ?
+WizardSelectDir=選擇目的資料夾
+SelectDirDesc=選擇安裝程式安裝 [name] 的位置。
+SelectDirLabel3=安裝程式將會把 [name] 安裝到下面的資料夾。
+SelectDirBrowseLabel=按 [下一步] 繼續,如果您想選擇另一個資料夾,請按 [瀏覽]。
+DiskSpaceMBLabel=最少需要 [mb] MB 磁碟空間。
+CannotInstallToNetworkDrive=安裝程式無法安裝於網絡磁碟機。
+CannotInstallToUNCPath=安裝程式無法安裝於 UNC 路徑。
+InvalidPath=您必須輸入完整的路徑名稱及磁碟機代碼。%n%n例如 C:\App 或 UNC 路徑格式 \\伺服器\共用資料夾。
+InvalidDrive=您選取的磁碟機或 UNC 名稱不存在或無法存取,請選擇其他的目的地。
+DiskSpaceWarningTitle=磁碟空間不足
+DiskSpaceWarning=安裝程式需要至少 %1 KB 的磁碟空間,您所選取的磁碟只有 %2 KB 可用空間。%n%n您要繼續安裝嗎?
+DirNameTooLong=資料夾名稱或路徑太長。
+InvalidDirName=資料夾名稱不正確。
+BadDirName32=資料夾名稱不得包含以下特殊字元:%n%n%1
+DirExistsTitle=資料夾已經存在
+DirExists=資料夾:%n%n%1%n%n 已經存在。仍要安裝到該資料夾嗎?
+DirDoesntExistTitle=資料夾不存在
+DirDoesntExist=資料夾:%n%n%1%n%n 不存在。要建立該資料夾嗎?
+
; *** "Select Components" wizard page
-WizardSelectComponents=
-SelectComponentsDesc=w˭Ǥ?
-SelectComponentsLabel2=znw˪; Mznw˪CzdzƦnn~ɡAЫ@U [U@B]C
-FullInstallation=w
+WizardSelectComponents=選擇元件
+SelectComponentsDesc=選擇將會被安裝的元件。
+SelectComponentsLabel2=選擇您想要安裝的元件;清除您不想安裝的元件。然後按 [下一步] 繼續安裝。
+FullInstallation=完整安裝
; if possible don't translate 'Compact' as 'Minimal' (I mean 'Minimal' in your language)
-CompactInstallation=²w
-CustomInstallation=ۭqw
-NoUninstallWarningTitle=w
-NoUninstallWarning=w˵{zqwwˤFUC:%n%n%1%n%nNoǤä|ϤѰwˡC%n%nn~?
+CompactInstallation=最小安裝
+CustomInstallation=自訂安裝
+NoUninstallWarningTitle=元件已存在
+NoUninstallWarning=安裝程式偵測到以下元件已經安裝在您的電腦上:%n%n%1%n%n取消選擇這些元件將不會移除它們。%n%n您仍然要繼續嗎?
ComponentSize1=%1 KB
ComponentSize2=%1 MB
-ComponentsDiskSpaceMBLabel=ثeܦܤֻݭn [mb] MB ϺЪŶC
+ComponentsDiskSpaceMBLabel=目前的選擇需要至少 [mb] MB 磁碟空間。
+
; *** "Select Additional Tasks" wizard page
-WizardSelectTasks=Lu@
-SelectTasksDesc=ٶǨLu@?
-SelectTasksLabel2=пw˵{bw [name] ɡAB~檺Lu@AM@U [U@B]C
+WizardSelectTasks=選擇附加的工作
+SelectTasksDesc=選擇要執行的附加工作。
+SelectTasksLabel2=選擇安裝程式在安裝 [name] 時要執行的附加工作,然後按 [下一步]。
+
; *** "Select Start Menu Folder" wizard page
-WizardSelectProgramGroup= [}l] \Ƨ
-SelectStartMenuFolderDesc=w˵{N{|mB?
-SelectStartMenuFolderLabel3=w˵{NbUC [}l] \Ƨإߵ{|C
-SelectStartMenuFolderBrowseLabel=Yn~AЫ@U [U@B]CYzQPƧAЫ@U [s]C
-MustEnterGroupName=JƧW١C
-GroupNameTooLong=ƧW٩θ|LC
-InvalidGroupName=ƧWٵLġC
-BadGroupName=ƧW٤o]tUC@r:%n%n%1
-NoProgramGroupCheck2=nإ [}l] \Ƨ(&D)
+WizardSelectProgramGroup=選擇「開始」功能表的資料夾
+SelectStartMenuFolderDesc=選擇安裝程式建立程式的捷徑的位置。
+SelectStartMenuFolderLabel3=安裝程式將會把程式的捷徑建立在下面的「開始」功能表資料夾。
+SelectStartMenuFolderBrowseLabel=按 [下一步] 繼續,如果您想選擇另一個資料夾,請按 [瀏覽]。
+MustEnterGroupName=您必須輸入一個資料夾的名稱。
+GroupNameTooLong=資料夾名稱或路徑太長。
+InvalidGroupName=資料夾名稱不正確。
+BadGroupName=資料夾名稱不得包含下列字元:%n%n%1
+NoProgramGroupCheck2=不要在「開始」功能表中建立資料夾(&D)
+
; *** "Ready to Install" wizard page
-WizardReady=wi}lw
-ReadyLabel1=w˵{{bwi}lN [name] w˨zqWC
-ReadyLabel2a=Yn~wˡAЫ@U [w]; Yn˾\ܧ]wAЫ@U [W@B]C
-ReadyLabel2b=Yn~wˡAЫ@U [w]C
-ReadyMemoUserInfo=ϥΪ̸T:
-ReadyMemoDir=تam:
-ReadyMemoType=w:
-ReadyMemoComponents=:
-ReadyMemoGroup=[}l] \Ƨ:
-ReadyMemoTasks=Lu@:
+WizardReady=準備安裝
+ReadyLabel1=安裝程式將開始安裝 [name] 到您的電腦中。
+ReadyLabel2a=按下 [安裝] 繼續安裝,或按 [上一步] 重新檢視或設定各選項的內容。
+ReadyLabel2b=按下 [安裝] 繼續安裝。
+ReadyMemoUserInfo=使用者資訊
+ReadyMemoDir=目的資料夾:
+ReadyMemoType=安裝型態:
+ReadyMemoComponents=選擇的元件:
+ReadyMemoGroup=「開始」功能表資料夾:
+ReadyMemoTasks=附加工作:
+
; *** "Preparing to Install" wizard page
-WizardPreparing=bdzƦw
-PreparingDesc=w˵{bdzƱN [name] w˨zqWC
-PreviousInstallNotCompleted=W@ӵ{w/|CsҰʹqA~৹ӦwˡC%n%nЦbsҰʹqAsw˵{AH [name] wˡC
-CannotContinue=w˵{Lk~CЫ@U [] HC
-ApplicationsFound=w˵{sUCε{bϥΪ@ɮסCijz\w˵{۰oε{C
-ApplicationsFound2=w˵{sUCε{bϥΪ@ɮסCijz\w˵{۰oε{Cw˧Aw˵{N|խsҰʳoε{C
-CloseApplications=۰ε{(&A)
-DontCloseApplications=nε{(&D)
-ErrorCloseApplications=w˵{Lk۰Ҧε{CijzҦbϥΦw˵{sɮתε{AMA~C
+WizardPreparing=準備安裝程式
+PreparingDesc=安裝程式準備將 [name] 安裝到您的電腦上。
+PreviousInstallNotCompleted=先前的安裝/ 解除安裝尚未完成,您必須重新啟動電腦以完成該安裝。%n%n在重新啟動電腦之後,請再執行這個程式來安裝 [name]。
+CannotContinue=安裝程式無法繼續。請按 [取消] 離開。
+ApplicationsFound=下面的應用程式正在使用安裝程式所需要更新的文檔。建議您允許安裝程式自動關閉這些應用程式。
+ApplicationsFound2=下面的應用程式正在使用安裝程式所需要更新的文檔。建議您允許安裝程式自動關閉這些應用程式。當安裝過程結束後,本安裝程式將會嘗試重新開啟該應用程式。
+CloseApplications=關閉應用程式(&A)
+DontCloseApplications=不要關閉應用程式 (&D)
+ErrorCloseApplications=安裝程式無法自動關閉所有應用程式。建議您在繼續前先關閉所有應用程式使用的檔案。
+
; *** "Installing" wizard page
-WizardInstalling=wˤ
-InstallingLabel=еyԡAw˵{bN [name] w˨zqWC
+WizardInstalling=正在安裝
+InstallingLabel=請稍候,安裝程式正在將 [name] 安裝到您的電腦上
+
; *** "Setup Completed" wizard page
-FinishedHeadingLabel=b [name] w˺F
-FinishedLabelNoIcons=w˵{wzqW [name] wˡC
-FinishedLabel=w˵{wzqW [name] wˡCziHҦw˪|ӱҰε{C
-ClickFinish=Ы@U []AHwˡC
-FinishedRestartLabel=w˵{sҰʱzqA~৹ [name] wˡCnߧYsҰʶ?
-FinishedRestartMessage=w˵{sҰʱzqA~৹ [name] wˡC%n%nnߧYsҰʶ?
-ShowReadmeCheck=OAڭn˵Ūɮ
-YesRadio=OAߧYsҰʹq(&Y)
-NoRadio=_AyԦAsҰʹq(&N)
+FinishedHeadingLabel=安裝完成
+FinishedLabelNoIcons=安裝程式已經將 [name] 安裝在您的電腦上。
+FinishedLabel=安裝程式已經將 [name] 安裝在您的電腦中,您可以選擇程式的圖示來執行該應用程式。
+ClickFinish=按 [完成] 以結束安裝程式。
+FinishedRestartLabel=要完成 [name] 的安裝,安裝程式必須重新啟動您的電腦。您想要現在重新啟動電腦嗎?
+FinishedRestartMessage=要完成 [name] 的安裝,安裝程式必須重新啟動您的電腦。%n%n您想要現在重新啟動電腦嗎?
+ShowReadmeCheck=是,我要閱讀讀我檔案。
+YesRadio=是,立即重新啟動電腦(&Y)
+NoRadio=否,我稍後重新啟動電腦(&N)
; used for example as 'Run MyProg.exe'
-RunEntryExec= %1
+RunEntryExec=執行 %1
; used for example as 'View Readme.txt'
-RunEntryShellExec=˵ %1
-; *** "Setup Needs the Next Disk" stuff
-ChangeDiskTitle=w˵{ݭnU@iϤC
-SelectDiskLabel2=дJϤ %1AM@U [Tw]C%n%nYϤWɮץiHbUCܤƧH~ƧAпJT|AΫ@U [s]C
-PathLabel=|(&P):
-FileNotInDir2=b "%2" 䤣ɮ "%1"CдJTϤAοLƧC
-SelectDirectoryLabel=ЫwU@iϤmC
+RunEntryShellExec=檢視 %1
+
+; *** "Setup Needs the Next Disk"
+ChangeDiskTitle=安裝程式需要下一張磁片
+SelectDiskLabel2=請插入磁片 %1,然後按 [確定]。%n%n如果檔案不在以下所顯示的資料夾之中,請輸入正確的資料夾名稱或按 [瀏覽] 選取。
+PathLabel=路徑(&P):
+FileNotInDir2=檔案“%1”無法在“%2”找到。請插入正確的磁片或選擇其它的資料夾。
+SelectDirectoryLabel=請指定下一張磁片的位置。
+
; *** Installation phase messages
-SetupAborted=w˥wC%n%nЭץDAAsw˵{C
-EntryAbortRetryIgnore=YnAդ@AЫ@U []; Yn~AЫ@U []; YnwˡAЫ@U []C
+SetupAborted=安裝沒有完成。%n%n請更正問題後重新安裝一次。
+AbortRetryIgnoreSelectAction=選取動作
+AbortRetryIgnoreRetry=請再試一次 (&T)
+AbortRetryIgnoreIgnore=略過錯誤並繼續 (&I)
+AbortRetryIgnoreCancel=取消安裝
+
; *** Installation status messages
-StatusClosingApplications=bε{...
-StatusCreateDirs=bإߥؿ...
-StatusExtractFiles=bYɮ...
-StatusCreateIcons=bإ߱|...
-StatusCreateIniEntries=bإ INI ...
-StatusCreateRegistryEntries=bإߵn...
-StatusRegisterFiles=bnɮ...
-StatusSavingUninstall=bxsѰw˸T...
-StatusRunProgram=bw...
-StatusRestartingApplications=bsҰε{...
-StatusRollback=b_ܧ...
+StatusClosingApplications=正在關閉應用程式...
+StatusCreateDirs=正在建立資料夾...
+StatusExtractFiles=正在解壓縮檔案...
+StatusCreateIcons=正在建立程式集圖示...
+StatusCreateIniEntries=寫入 INI 檔案的項目...
+StatusCreateRegistryEntries=正在更新系統登錄...
+StatusRegisterFiles=正在登錄檔案...
+StatusSavingUninstall=儲存解除安裝資訊...
+StatusRunProgram=正在完成安裝...
+StatusRestartingApplications=正在重新開啟應用程式...
+StatusRollback=正在復原變更...
+
; *** Misc. errors
-ErrorInternal2=~: %1
-ErrorFunctionFailedNoCode=%1
-ErrorFunctionFailed=%1 ; NX %2
-ErrorFunctionFailedWithMessage=%1 ; NX %2C%n%3
-ErrorExecutingProgram=Lkɮ:%n%1
+ErrorInternal2=內部錯誤: %1
+ErrorFunctionFailedNoCode=%1 失敗
+ErrorFunctionFailed=%1 失敗;代碼 %2
+ErrorFunctionFailedWithMessage=%1 失敗;代碼 %2.%n%3
+ErrorExecutingProgram=無法執行檔案:%n%1
+
; *** Registry errors
-ErrorRegOpenKey=}ҵnXɵoͿ~:%n%1\%2
-ErrorRegCreateKey=إߵnXɵoͿ~:%n%1\%2
-ErrorRegWriteKey=gJnXɵoͿ~:%n%1\%2
+ErrorRegOpenKey=無法開啟登錄鍵:%n%1\%2
+ErrorRegCreateKey=無法建立登錄項目:%n%1\%2
+ErrorRegWriteKey=無法變更登錄項目:%n%1\%2
+
; *** INI errors
-ErrorIniEntry=bɮ "%1" إ INI خɵoͿ~C
+ErrorIniEntry=在檔案“%1”建立 INI 項目錯誤。
+
; *** File copying errors
-FileAbortRetryIgnore=YnAդ@AЫ@U []; YnLɮסAЫ@U [] (ijϥ); YnwˡAЫ@U []C
-FileAbortRetryIgnore2=YnAդ@AЫ@U []; Yn~AЫ@U [] (ijϥ); YnwˡAЫ@U []C
-SourceIsCorrupted=l{ɤwl
-SourceDoesntExist=l{ "%1" sb
-ExistingFileReadOnly={ɮפwаOŪC%n%nYnŪݩʡAMAդ@AЫ@U []; YnLɮסAЫ@U []; YnwˡAЫ@U []C
-ErrorReadingExistingDest=Ū{ɮɵoͿ~:
-FileExists=wɮסC%n%nnѦw˵{[Hмg?
-ExistingFileNewer={ɮw˵{զw˪ɮsCijzOd{ɮסC%n%nnOd{ɮ?
-ErrorChangingAttr=ܧ{ɮתݩʮɵoͿ~:
-ErrorCreatingTemp=զbتaؿإɮɵoͿ~:
-ErrorReadingSource=Ūl{ɮɵoͿ~:
-ErrorCopying=սƻsɮɵoͿ~:
-ErrorReplacingExistingFile=ըN{ɮɵoͿ~:
-ErrorRestartReplace=RestartReplace :
-ErrorRenamingTemp=խsRWتaؿɮɵoͿ~:
-ErrorRegisterServer=Lkn DLL/OCX: %1
-ErrorRegSvr32Failed=RegSvr32 ѡANX %1
-ErrorRegisterTypeLib=Lkn{w: %1
+FileAbortRetryIgnoreSkipNotRecommended=略過這個檔案 (不建議) (&S)
+FileAbortRetryIgnoreIgnoreNotRecommended=略過錯誤並繼續 (不建議) (&I)
+SourceDoesntExist=來源檔案“%1”不存在。
+SourceIsCorrupted=來源檔案已經損毀。
+ExistingFileReadOnly2=無法取代現有檔案,因為檔案已標示為唯讀。
+ExistingFileReadOnlyRetry=移除唯讀屬性並重試 (&R)
+ExistingFileReadOnlyKeepExisting=保留現有檔案 (&K)
+ErrorReadingExistingDest=讀取一個已存在的檔案時發生錯誤:
+FileExists=檔案已經存在。%n%n 要讓安裝程式加以覆寫嗎?
+ExistingFileNewer=存在的檔案版本比較新,建議您保留目前已存在的檔案。%n%n您要保留目前已存在的檔案嗎?
+ErrorChangingAttr=在變更檔案屬性時發生錯誤:
+ErrorCreatingTemp=在目的資料夾中建立檔案時發生錯誤:
+ErrorReadingSource=讀取原始檔案時發生錯誤:
+ErrorCopying=復制檔案時發生錯誤:
+ErrorReplacingExistingFile=取代檔案時發生錯誤:
+ErrorRestartReplace=重新啟動電腦後取代檔案失敗:
+ErrorRenamingTemp=在目的資料夾變更檔案名稱時發生錯誤:
+ErrorRegisterServer=無法注冊 DLL/OCX 檔案: %1。
+ErrorRegSvr32Failed=RegSvr32 失敗;退出代碼 %1
+ErrorRegisterTypeLib=無法注冊類型庫: %1。
+
+; *** Uninstall display name markings
+; used for example as 'My Program (32-bit)'
+UninstallDisplayNameMark=%1 (%2)
+; used for example as 'My Program (32-bit, All users)'
+UninstallDisplayNameMarks=%1 (%2, %3)
+UninstallDisplayNameMark32Bit=32-bit
+UninstallDisplayNameMark64Bit=64-bit
+UninstallDisplayNameMarkAllUsers=所有使用者
+UninstallDisplayNameMarkCurrentUser=目前使用者
+
; *** Post-installation errors
-ErrorOpeningReadme=ն}ŪɮɵoͿ~C
-ErrorRestartingComputer=w˵{LksҰʹqCФʰ榹@~C
+ErrorOpeningReadme=開啟讀我檔案時發生錯誤。
+ErrorRestartingComputer=安裝程式無法重新啟動電腦,請以手動方式自行重新啟動電腦。
+
; *** Uninstaller messages
-UninstallNotFound=Sɮ "%1"CLkѰwˡC
-UninstallOpenError=Lk}ɮ "%1"CLkѰw
-UninstallUnsupportedVer=Ѱw˵{LkѸѰw˰O "%1" 榡CLkѰw
-UninstallUnknownEntry=bѰw˰O줣 (%1)
-ConfirmUninstall=Twn %1 ΨҦ?
-UninstallOnlyOnWin64=uib 64 줸 Windows WѰw˦wˡC
-OnlyAdminCanUninstall=uƨtκzvϥΪ̡A~Ѱw˦wˡC
-UninstallStatusLabel=bqzq %1AеyԡC
-UninstalledAll=w\qzq %1C
-UninstalledMost=Ѱw %1 wC%n%nصLkCziHʥ[HC
-UninstalledAndNeedsRestart=Yn %1 ѰwˡAsҰʱzqC%n%nnߧYsҰʶ?
-UninstallDataCorrupted="%1" ɮפwlCLkѰw
+UninstallNotFound=檔案“%1”不存在,無法移除程式。
+UninstallOpenError=無法開啟檔案“%1”,無法移除程式。
+UninstallUnsupportedVer=這個版本的解除安裝程式無法辨識記錄檔 “%1” 之格式,無法解除安裝。
+UninstallUnknownEntry=解除安裝記錄檔中發現未知的記錄 (%1)。
+ConfirmUninstall=您確定要完全移除 %1 及其相關的檔案嗎?
+UninstallOnlyOnWin64=這個程式只能在 64 位元的 Windows 上解除安裝。
+OnlyAdminCanUninstall=這個程式要具備系統管理員權限的使用者方可解除安裝。
+UninstallStatusLabel=正在從您的電腦移除 %1 中,請稍候...
+UninstalledAll=%1 已經成功從您的電腦中移除。
+UninstalledMost=%1 解除安裝完成。%n%n某些檔案及元件無法移除,您可以自行刪除這些檔案。
+UninstalledAndNeedsRestart=要完成 %1 的解除安裝程序,您必須重新啟動電腦。%n%n您想要現在重新啟動電腦嗎?
+UninstallDataCorrupted=檔案“%1”已經損毀,無法解除安裝。
+
; *** Uninstallation phase messages
-ConfirmDeleteSharedFileTitle=n@ɮ?
-ConfirmDeleteSharedFile2=tΫXwL{bϥΤUC@ɮסCznѰwˡAH@ɮ?%n%np{bϥΦɮצӱNɮײAoǵ{iLk`B@CYTwAп [_]CNɮOdbtΤWä|y}vTC
-SharedFileNameLabel=ɮצW:
-SharedFileLocationLabel=m:
-WizardUninstalling=Ѱw˪A
-StatusUninstalling=bѰw %1...
+ConfirmDeleteSharedFileTitle=移除共用檔案
+ConfirmDeleteSharedFile2=系統顯示下列共用檔案已不再被任何程式所使用,您要移除這些檔案嗎?%n%n%1%n%n倘若您移除了以上檔案但仍有程式需要使用它們,將造成這些程式無法正常執行,因此您若無法確定請選擇 [否]。保留這些檔案在您的系統中不會造成任何損害。
+SharedFileNameLabel=檔案名稱:
+SharedFileLocationLabel=位置:
+WizardUninstalling=解除安裝狀態
+StatusUninstalling=正在解除安裝 %1...
+
; *** Shutdown block reasons
-ShutdownBlockReasonInstallingApp=bw %1C
-ShutdownBlockReasonUninstallingApp=bѰw %1C
+ShutdownBlockReasonInstallingApp=正在安裝 %1.
+ShutdownBlockReasonUninstallingApp=正在解除安裝 %1.
+
; The custom messages below aren't used by Setup itself, but if you make
; use of them in your scripts, you'll want to translate them.
+
[CustomMessages]
-NameAndVersion=%1 %2
-AdditionalIcons=L|:
-CreateDesktopIcon=إ߮ୱ|(&D)
-CreateQuickLaunchIcon=إߧֳtҰʱ|(&Q)
-ProgramOnTheWeb=Web W %1
-UninstallProgram=Ѱw %1
-LaunchProgram=Ұ %1
-AssocFileExtension=p %1 P %2 ɦW(&A)
-AssocingFileExtension=bإ %1 P %2 ɦWpK
-AutoStartProgramGroupDescription=Ұ:
-AutoStartProgram=۰ʱҰ %1
-AddonHostProgramNotFound=bƧ䤣 %1C%n%nn~?
\ No newline at end of file
+
+NameAndVersion=%1 版本 %2
+AdditionalIcons=附加圖示:
+CreateDesktopIcon=建立桌面圖示(&D)
+CreateQuickLaunchIcon=建立快速啟動圖示(&Q)
+ProgramOnTheWeb=%1 的網站
+UninstallProgram=解除安裝 %1
+LaunchProgram=啟動 %1
+AssocFileExtension=將 %1 與檔案副檔名 %2 產生關聯(&A)
+AssocingFileExtension=正在將 %1 與檔案副檔名 %2 產生關聯...
+AutoStartProgramGroupDescription=開啟:
+AutoStartProgram=自動開啟 %1
+AddonHostProgramNotFound=%1 無法在您所選的資料夾中找到。%n%n您是否還要繼續?
diff --git a/build/win32/i18n/messages.de.isl b/build/win32/i18n/messages.de.isl
index 755652bcdbe95..6a9f29aa9c27e 100644
--- a/build/win32/i18n/messages.de.isl
+++ b/build/win32/i18n/messages.de.isl
@@ -5,4 +5,5 @@ AssociateWithFiles=%1 als Editor f
AddToPath=Zu PATH hinzufgen (nach dem Neustart verfgbar)
RunAfter=%1 nach der Installation ausfhren
Other=Andere:
-SourceFile=%1-Quelldatei
\ No newline at end of file
+SourceFile=%1-Quelldatei
+OpenWithCodeContextMenu=Mit %1 ffnen
\ No newline at end of file
diff --git a/build/win32/i18n/messages.en.isl b/build/win32/i18n/messages.en.isl
index a6aab59b95a97..986eba00d3e58 100644
--- a/build/win32/i18n/messages.en.isl
+++ b/build/win32/i18n/messages.en.isl
@@ -1,8 +1,16 @@
+[Messages]
+FinishedLabel=Setup has finished installing [name] on your computer. The application may be launched by selecting the installed shortcuts.
+ConfirmUninstall=Are you sure you want to completely remove %1 and all of its components?
+
[CustomMessages]
+AdditionalIcons=Additional icons:
+CreateDesktopIcon=Create a &desktop icon
+CreateQuickLaunchIcon=Create a &Quick Launch icon
AddContextMenuFiles=Add "Open with %1" action to Windows Explorer file context menu
AddContextMenuFolders=Add "Open with %1" action to Windows Explorer directory context menu
AssociateWithFiles=Register %1 as an editor for supported file types
AddToPath=Add to PATH (requires shell restart)
RunAfter=Run %1 after installation
Other=Other:
-SourceFile=%1 Source File
\ No newline at end of file
+SourceFile=%1 Source File
+OpenWithCodeContextMenu=Open w&ith %1
diff --git a/build/win32/i18n/messages.es.isl b/build/win32/i18n/messages.es.isl
index e8d5af64b6193..e51f099f9a136 100644
--- a/build/win32/i18n/messages.es.isl
+++ b/build/win32/i18n/messages.es.isl
@@ -5,4 +5,5 @@ AssociateWithFiles=Registrar %1 como editor para tipos de archivo admitidos
AddToPath=Agregar a PATH (disponible despus de reiniciar)
RunAfter=Ejecutar %1 despus de la instalacin
Other=Otros:
-SourceFile=Archivo de origen %1
\ No newline at end of file
+SourceFile=Archivo de origen %1
+OpenWithCodeContextMenu=Abrir con %1
\ No newline at end of file
diff --git a/build/win32/i18n/messages.fr.isl b/build/win32/i18n/messages.fr.isl
index 3e17036f80673..df14041862513 100644
--- a/build/win32/i18n/messages.fr.isl
+++ b/build/win32/i18n/messages.fr.isl
@@ -5,4 +5,5 @@ AssociateWithFiles=Inscrire %1 en tant qu'
AddToPath=Ajouter PATH (disponible aprs le redmarrage)
RunAfter=Excuter %1 aprs l'installation
Other=Autre:
-SourceFile=Fichier source %1
\ No newline at end of file
+SourceFile=Fichier source %1
+OpenWithCodeContextMenu=Ouvrir avec %1
\ No newline at end of file
diff --git a/build/win32/i18n/messages.hu.isl b/build/win32/i18n/messages.hu.isl
index f141ad1b24ad6..b64553da8e6de 100644
--- a/build/win32/i18n/messages.hu.isl
+++ b/build/win32/i18n/messages.hu.isl
@@ -5,4 +5,5 @@ AssociateWithFiles=%1 regisztr
AddToPath=Hozzads a PATH-hoz (jraindts utn lesz elrhet)
RunAfter=%1 indtsa a telepts utn
Other=Egyb:
-SourceFile=%1 forrsfjl
\ No newline at end of file
+SourceFile=%1 forrsfjl
+OpenWithCodeContextMenu=Megnyits a kvetkezvel: %1
\ No newline at end of file
diff --git a/build/win32/i18n/messages.it.isl b/build/win32/i18n/messages.it.isl
index 7ad8a0622d45a..08248c4ce1ba8 100644
--- a/build/win32/i18n/messages.it.isl
+++ b/build/win32/i18n/messages.it.isl
@@ -5,4 +5,5 @@ AssociateWithFiles=Registra %1 come editor per i tipi di file supportati
AddToPath=Aggiungi a PATH (disponibile dopo il riavvio)
RunAfter=Esegui %1 dopo l'installazione
Other=Altro:
-SourceFile=File di origine %1
\ No newline at end of file
+SourceFile=File di origine %1
+OpenWithCodeContextMenu=Apri con %1
\ No newline at end of file
diff --git a/build/win32/i18n/messages.ja.isl b/build/win32/i18n/messages.ja.isl
index 3a16aaa204e97..9675060e94afd 100644
--- a/build/win32/i18n/messages.ja.isl
+++ b/build/win32/i18n/messages.ja.isl
@@ -5,4 +5,5 @@ AssociateWithFiles=
AddToPath=PATH ւ̒ljiċNɎgp\j
RunAfter=CXg[ %1 s
Other=̑:
-SourceFile=%1 \[X t@C
\ No newline at end of file
+SourceFile=%1 \[X t@C
+OpenWithCodeContextMenu=%1 ŊJ
\ No newline at end of file
diff --git a/build/win32/i18n/messages.ko.isl b/build/win32/i18n/messages.ko.isl
index 28860c36b633e..5a510558bbd2b 100644
--- a/build/win32/i18n/messages.ko.isl
+++ b/build/win32/i18n/messages.ko.isl
@@ -5,4 +5,5 @@ AssociateWithFiles=%1
AddToPath=PATH ߰(ٽ )
RunAfter=ġ %1
Other=Ÿ:
-SourceFile=%1
\ No newline at end of file
+SourceFile=%1
+OpenWithCodeContextMenu=%1()
\ No newline at end of file
diff --git a/build/win32/i18n/messages.pt-br.isl b/build/win32/i18n/messages.pt-br.isl
index d534637f8b613..e327e8fd1a066 100644
--- a/build/win32/i18n/messages.pt-br.isl
+++ b/build/win32/i18n/messages.pt-br.isl
@@ -5,4 +5,5 @@ AssociateWithFiles=Registre %1 como um editor para tipos de arquivos suportados
AddToPath=Adicione em PATH (disponvel aps reiniciar)
RunAfter=Executar %1 aps a instalao
Other=Outros:
-SourceFile=Arquivo Fonte %1
\ No newline at end of file
+SourceFile=Arquivo Fonte %1
+OpenWithCodeContextMenu=Abrir com %1
\ No newline at end of file
diff --git a/build/win32/i18n/messages.ru.isl b/build/win32/i18n/messages.ru.isl
index 4d834663627e1..bca3b864a5f9e 100644
--- a/build/win32/i18n/messages.ru.isl
+++ b/build/win32/i18n/messages.ru.isl
@@ -5,4 +5,5 @@ AssociateWithFiles=
AddToPath= PATH ( )
RunAfter= %1
Other=:
-SourceFile= %1
\ No newline at end of file
+SourceFile= %1
+OpenWithCodeContextMenu= %1
\ No newline at end of file
diff --git a/build/win32/i18n/messages.tr.isl b/build/win32/i18n/messages.tr.isl
index dc241d924c7bc..b13e5e27bd2af 100644
--- a/build/win32/i18n/messages.tr.isl
+++ b/build/win32/i18n/messages.tr.isl
@@ -5,4 +5,5 @@ AssociateWithFiles=%1 uygulamas
AddToPath=PATH'e ekle (yeniden balattktan sonra kullanlabilir)
RunAfter=Kurulumdan sonra %1 uygulamasn altr.
Other=Dier:
-SourceFile=%1 Kaynak Dosyas
\ No newline at end of file
+SourceFile=%1 Kaynak Dosyas
+OpenWithCodeContextMenu=%1 le A
\ No newline at end of file
diff --git a/build/win32/i18n/messages.zh-cn.isl b/build/win32/i18n/messages.zh-cn.isl
index 349fc2ccc29b5..8fa136f6d5a9b 100644
--- a/build/win32/i18n/messages.zh-cn.isl
+++ b/build/win32/i18n/messages.zh-cn.isl
@@ -5,4 +5,5 @@ AssociateWithFiles=
AddToPath=ӵ PATH (Ч)
RunAfter=װ %1
Other=:
-SourceFile=%1 Դļ
\ No newline at end of file
+SourceFile=%1 Դļ
+OpenWithCodeContextMenu=ͨ %1
\ No newline at end of file
diff --git a/build/win32/i18n/messages.zh-tw.isl b/build/win32/i18n/messages.zh-tw.isl
index 7c3f84aa131f5..40c5fa92d793e 100644
--- a/build/win32/i18n/messages.zh-tw.isl
+++ b/build/win32/i18n/messages.zh-tw.isl
@@ -5,4 +5,5 @@ AssociateWithFiles=
AddToPath=[J PATH (sҰʫͮ)
RunAfter=w˫ %1
Other=L:
-SourceFile=%1 ӷɮ
\ No newline at end of file
+SourceFile=%1 ӷɮ
+OpenWithCodeContextMenu=H %1 }
\ No newline at end of file
diff --git a/build/yarn.lock b/build/yarn.lock
index 36f6c025d487c..01ebd186c4cbc 100644
--- a/build/yarn.lock
+++ b/build/yarn.lock
@@ -90,6 +90,24 @@
resolved "https://registry.yarnpkg.com/@types/debug/-/debug-4.1.5.tgz#b14efa8852b7768d898906613c23f688713e02cd"
integrity sha512-Q1y515GcOdTHgagaVFhHnIFQ38ygs/kmxdNpvpou+raI9UO3YZcHDngBSYKQklcKlvA7iuQlmIKbzvmxcOE9CQ==
+"@types/eslint-visitor-keys@^1.0.0":
+ version "1.0.0"
+ resolved "https://registry.yarnpkg.com/@types/eslint-visitor-keys/-/eslint-visitor-keys-1.0.0.tgz#1ee30d79544ca84d68d4b3cdb0af4f205663dd2d"
+ integrity sha512-OCutwjDZ4aFS6PB1UZ988C4YgwlBHJd6wCeQqaLdmadZ/7e+w79+hbMUFC1QXDNCmdyoRfAFdm0RypzwR+Qpag==
+
+"@types/eslint@4.16.1":
+ version "4.16.1"
+ resolved "https://registry.yarnpkg.com/@types/eslint/-/eslint-4.16.1.tgz#19730c9fcb66b6e44742d12b27a603fabfeb2f49"
+ integrity sha512-lRUXQAULl5geixTiP2K0iYvMUbCkEnuOwvLGjwff12I4ECxoW5QaWML5UUOZ1CvpQLILkddBdMPMZz4ByQizsg==
+ dependencies:
+ "@types/estree" "*"
+ "@types/json-schema" "*"
+
+"@types/estree@*":
+ version "0.0.41"
+ resolved "https://registry.yarnpkg.com/@types/estree/-/estree-0.0.41.tgz#fd90754150b57432b72bf560530500597ff04421"
+ integrity sha512-rIAmXyJlqw4KEBO7+u9gxZZSQHaCNnIzYrnNmYVpgfJhxTqO0brCX0SYpqUTkVI5mwwUwzmtspLBGBKroMeynA==
+
"@types/events@*":
version "1.2.0"
resolved "https://registry.yarnpkg.com/@types/events/-/events-1.2.0.tgz#81a6731ce4df43619e5c8c945383b3e62a89ea86"
@@ -184,6 +202,11 @@
resolved "https://registry.yarnpkg.com/@types/js-beautify/-/js-beautify-1.8.0.tgz#0369d3d0e1f35a6aec07cb4da2ee2bcda111367c"
integrity sha512-/siF86XrwDKLuHe8l7h6NhrAWgLdgqbxmjZv9NvGWmgYRZoTipkjKiWb0SQHy/jcR+ee0GvbG6uGd+LEBMGNvA==
+"@types/json-schema@*", "@types/json-schema@^7.0.3":
+ version "7.0.4"
+ resolved "https://registry.yarnpkg.com/@types/json-schema/-/json-schema-7.0.4.tgz#38fd73ddfd9b55abb1e1b2ed578cb55bd7b7d339"
+ integrity sha512-8+KAKzEvSUdeo+kmqnKrqgeE+LcA0tjYWFY7RPProVYwnqDjukzO+3b6dLD56rYX5TdWejnEOLJYOIeh4CXKuA==
+
"@types/mime@0.0.29":
version "0.0.29"
resolved "https://registry.yarnpkg.com/@types/mime/-/mime-0.0.29.tgz#fbcfd330573b912ef59eeee14602bface630754b"
@@ -312,11 +335,70 @@
resolved "https://registry.yarnpkg.com/@types/xml2js/-/xml2js-0.0.33.tgz#20c5dd6460245284d64a55690015b95e409fb7de"
integrity sha1-IMXdZGAkUoTWSlVpABW5XkCft94=
+"@typescript-eslint/experimental-utils@2.14.0":
+ version "2.14.0"
+ resolved "https://registry.yarnpkg.com/@typescript-eslint/experimental-utils/-/experimental-utils-2.14.0.tgz#e9179fa3c44e00b3106b85d7b69342901fb43e3b"
+ integrity sha512-KcyKS7G6IWnIgl3ZpyxyBCxhkBPV+0a5Jjy2g5HxlrbG2ZLQNFeneIBVXdaBCYOVjvGmGGFKom1kgiAY75SDeQ==
+ dependencies:
+ "@types/json-schema" "^7.0.3"
+ "@typescript-eslint/typescript-estree" "2.14.0"
+ eslint-scope "^5.0.0"
+
+"@typescript-eslint/experimental-utils@~2.13.0":
+ version "2.13.0"
+ resolved "https://registry.yarnpkg.com/@typescript-eslint/experimental-utils/-/experimental-utils-2.13.0.tgz#958614faa6f77599ee2b241740e0ea402482533d"
+ integrity sha512-+Hss3clwa6aNiC8ZjA45wEm4FutDV5HsVXPl/rDug1THq6gEtOYRGLqS3JlTk7mSnL5TbJz0LpEbzbPnKvY6sw==
+ dependencies:
+ "@types/json-schema" "^7.0.3"
+ "@typescript-eslint/typescript-estree" "2.13.0"
+ eslint-scope "^5.0.0"
+
+"@typescript-eslint/parser@^2.12.0":
+ version "2.14.0"
+ resolved "https://registry.yarnpkg.com/@typescript-eslint/parser/-/parser-2.14.0.tgz#30fa0523d86d74172a5e32274558404ba4262cd6"
+ integrity sha512-haS+8D35fUydIs+zdSf4BxpOartb/DjrZ2IxQ5sR8zyGfd77uT9ZJZYF8+I0WPhzqHmfafUBx8MYpcp8pfaoSA==
+ dependencies:
+ "@types/eslint-visitor-keys" "^1.0.0"
+ "@typescript-eslint/experimental-utils" "2.14.0"
+ "@typescript-eslint/typescript-estree" "2.14.0"
+ eslint-visitor-keys "^1.1.0"
+
+"@typescript-eslint/typescript-estree@2.13.0":
+ version "2.13.0"
+ resolved "https://registry.yarnpkg.com/@typescript-eslint/typescript-estree/-/typescript-estree-2.13.0.tgz#a2e746867da772c857c13853219fced10d2566bc"
+ integrity sha512-t21Mg5cc8T3ADEUGwDisHLIubgXKjuNRbkpzDMLb7/JMmgCe/gHM9FaaujokLey+gwTuLF5ndSQ7/EfQqrQx4g==
+ dependencies:
+ debug "^4.1.1"
+ eslint-visitor-keys "^1.1.0"
+ glob "^7.1.6"
+ is-glob "^4.0.1"
+ lodash.unescape "4.0.1"
+ semver "^6.3.0"
+ tsutils "^3.17.1"
+
+"@typescript-eslint/typescript-estree@2.14.0":
+ version "2.14.0"
+ resolved "https://registry.yarnpkg.com/@typescript-eslint/typescript-estree/-/typescript-estree-2.14.0.tgz#c67698acdc14547f095eeefe908958d93e1a648d"
+ integrity sha512-pnLpUcMNG7GfFFfNQbEX6f1aPa5fMnH2G9By+A1yovYI4VIOK2DzkaRuUlIkbagpAcrxQHLqovI1YWqEcXyRnA==
+ dependencies:
+ debug "^4.1.1"
+ eslint-visitor-keys "^1.1.0"
+ glob "^7.1.6"
+ is-glob "^4.0.1"
+ lodash.unescape "4.0.1"
+ semver "^6.3.0"
+ tsutils "^3.17.1"
+
acorn@4.X:
version "4.0.13"
resolved "https://registry.yarnpkg.com/acorn/-/acorn-4.0.13.tgz#105495ae5361d697bd195c825192e1ad7f253787"
integrity sha1-EFSVrlNh1pe9GVyCUZLhrX8lN4c=
+agent-base@5:
+ version "5.1.1"
+ resolved "https://registry.yarnpkg.com/agent-base/-/agent-base-5.1.1.tgz#e8fb3f242959db44d63be665db7a8e739537a32c"
+ integrity sha512-TMeqbNl2fMW0nMjTEPOwe3J/PRFP4vqeoNuQMG0HlMrtm5QxKqdvAkZ1pRBQ/ulIyDD5Yq0nJ7YbdD8ey0TO3g==
+
ajv@^4.9.1:
version "4.11.8"
resolved "https://registry.yarnpkg.com/ajv/-/ajv-4.11.8.tgz#82ffb02b29e662ae53bdc20af15947706739c536"
@@ -352,13 +434,6 @@ ansi-styles@^2.2.1:
resolved "https://registry.yarnpkg.com/ansi-styles/-/ansi-styles-2.2.1.tgz#b432dd3358b634cf75e1e4664368240533c1ddbe"
integrity sha1-tDLdM1i2NM914eRmQ2gkBTPB3b4=
-ansi-styles@^3.2.1:
- version "3.2.1"
- resolved "https://registry.yarnpkg.com/ansi-styles/-/ansi-styles-3.2.1.tgz#41fbb20243e50b12be0f04b8dedbf07520ce841d"
- integrity sha512-VT0ZI6kZRdTh8YyJw3SMbYm/u+NqfsAxEpWO0Pf9sq8/e94WxxOpPKx9FR1FlyCtOVDNOQ+8ntlqFxiRc+r5qA==
- dependencies:
- color-convert "^1.9.0"
-
ansi-wrap@0.1.0:
version "0.1.0"
resolved "https://registry.yarnpkg.com/ansi-wrap/-/ansi-wrap-0.1.0.tgz#a82250ddb0015e9a27ca82e82ea603bbfa45efaf"
@@ -482,20 +557,16 @@ azure-storage@^2.1.0:
xml2js "0.2.7"
xmlbuilder "0.4.3"
-babel-code-frame@^6.22.0:
- version "6.26.0"
- resolved "https://registry.yarnpkg.com/babel-code-frame/-/babel-code-frame-6.26.0.tgz#63fd43f7dc1e3bb7ce35947db8fe369a3f58c74b"
- integrity sha1-Y/1D99weO7fONZR9uP42mj9Yx0s=
- dependencies:
- chalk "^1.1.3"
- esutils "^2.0.2"
- js-tokens "^3.0.2"
-
balanced-match@^1.0.0:
version "1.0.0"
resolved "https://registry.yarnpkg.com/balanced-match/-/balanced-match-1.0.0.tgz#89b4d199ab2bee49de164ea02b89ce462d71b767"
integrity sha1-ibTRmasr7kneFk6gK4nORi1xt2c=
+base64-js@^1.2.3:
+ version "1.3.1"
+ resolved "https://registry.yarnpkg.com/base64-js/-/base64-js-1.3.1.tgz#58ece8cb75dd07e71ed08c736abc5fac4dbf8df1"
+ integrity sha512-mLQ4i2QO1ytvGWFWmcngKO//JXAQueZvwEKtjgQFM4jIK0kU+ytMfplL8j+n5mspOfjHwoAg+9yhb7BwAHm36g==
+
bcrypt-pbkdf@^1.0.0:
version "1.0.1"
resolved "https://registry.yarnpkg.com/bcrypt-pbkdf/-/bcrypt-pbkdf-1.0.1.tgz#63bc5dcb61331b92bc05fd528953c33462a06f8d"
@@ -508,6 +579,11 @@ beeper@^1.0.0:
resolved "https://registry.yarnpkg.com/beeper/-/beeper-1.1.1.tgz#e6d5ea8c5dad001304a70b22638447f69cb2f809"
integrity sha1-5tXqjF2tABMEpwsiY4RH9pyy+Ak=
+bluebird@^3.5.0:
+ version "3.7.2"
+ resolved "https://registry.yarnpkg.com/bluebird/-/bluebird-3.7.2.tgz#9f229c15be272454ffa973ace0dbee79a1b0c36f"
+ integrity sha512-XpNj6GDQzdfW+r2Wnn7xiSAd7TM3jzkxGXBGTtWKuSXv1xUV+azxAm8jdWZN06QTQk+2N2XB9jRDkvbmQmcRtg==
+
boolbase@~1.0.0:
version "1.0.0"
resolved "https://registry.yarnpkg.com/boolbase/-/boolbase-1.0.0.tgz#68dff5fbe60c51eb37725ea9e3ed310dcc1e776e"
@@ -554,27 +630,40 @@ browserify-mime@~1.2.9:
resolved "https://registry.yarnpkg.com/browserify-mime/-/browserify-mime-1.2.9.tgz#aeb1af28de6c0d7a6a2ce40adb68ff18422af31f"
integrity sha1-rrGvKN5sDXpqLOQK22j/GEIq8x8=
+buffer-alloc-unsafe@^1.1.0:
+ version "1.1.0"
+ resolved "https://registry.yarnpkg.com/buffer-alloc-unsafe/-/buffer-alloc-unsafe-1.1.0.tgz#bd7dc26ae2972d0eda253be061dba992349c19f0"
+ integrity sha512-TEM2iMIEQdJ2yjPJoSIsldnleVaAk1oW3DBVUykyOLsEsFmEc9kn+SFFPz+gl54KQNxlDnAwCXosOS9Okx2xAg==
+
+buffer-alloc@^1.2.0:
+ version "1.2.0"
+ resolved "https://registry.yarnpkg.com/buffer-alloc/-/buffer-alloc-1.2.0.tgz#890dd90d923a873e08e10e5fd51a57e5b7cce0ec"
+ integrity sha512-CFsHQgjtW1UChdXgbyJGtnm+O/uLQeZdtbDo8mfUgYXCHSM1wgrVxXm6bSyrUuErEb+4sYVGCzASBRot7zyrow==
+ dependencies:
+ buffer-alloc-unsafe "^1.1.0"
+ buffer-fill "^1.0.0"
+
buffer-crc32@~0.2.3:
version "0.2.13"
resolved "https://registry.yarnpkg.com/buffer-crc32/-/buffer-crc32-0.2.13.tgz#0d333e3f00eac50aa1454abd30ef8c2a5d9a7242"
integrity sha1-DTM+PwDqxQqhRUq9MO+MKl2ackI=
+buffer-fill@^1.0.0:
+ version "1.0.0"
+ resolved "https://registry.yarnpkg.com/buffer-fill/-/buffer-fill-1.0.0.tgz#f8f78b76789888ef39f205cd637f68e702122b2c"
+ integrity sha1-+PeLdniYiO858gXNY39o5wISKyw=
+
buffer-from@^1.0.0:
version "1.1.1"
resolved "https://registry.yarnpkg.com/buffer-from/-/buffer-from-1.1.1.tgz#32713bc028f75c02fdb710d7c7bcec1f2c6070ef"
integrity sha512-MQcXEUbCKtEo7bhqEs6560Hyd4XaovZlO/k9V3hjVUF/zwW7KBVdSK4gIt/bzwS9MbR5qob+F5jusZsb0YQK2A==
-builtin-modules@^1.1.1:
- version "1.1.1"
- resolved "https://registry.yarnpkg.com/builtin-modules/-/builtin-modules-1.1.1.tgz#270f076c5a72c02f5b65a47df94c5fe3a278892f"
- integrity sha1-Jw8HbFpywC9bZaR9+Uxf46J4iS8=
-
caseless@~0.12.0:
version "0.12.0"
resolved "https://registry.yarnpkg.com/caseless/-/caseless-0.12.0.tgz#1b681c21ff84033c826543090689420d187151dc"
integrity sha1-G2gcIf+EAzyCZUMJBolCDRhxUdw=
-chalk@^1.0.0, chalk@^1.1.3:
+chalk@^1.0.0:
version "1.1.3"
resolved "https://registry.yarnpkg.com/chalk/-/chalk-1.1.3.tgz#a8115c55e4a702fe4d150abd3872822a7e09fc98"
integrity sha1-qBFcVeSnAv5NFQq9OHKCKn4J/Jg=
@@ -585,15 +674,6 @@ chalk@^1.0.0, chalk@^1.1.3:
strip-ansi "^3.0.0"
supports-color "^2.0.0"
-chalk@^2.3.0:
- version "2.4.1"
- resolved "https://registry.yarnpkg.com/chalk/-/chalk-2.4.1.tgz#18c49ab16a037b6eb0152cc83e3471338215b66e"
- integrity sha512-ObN6h1v2fTJSmUXoS3nMQ92LbDK9be4TV+6G+omQlGJFdcUX5heKi1LZ1YnRMIgwTLEj3E24bT6tYni50rlCfQ==
- dependencies:
- ansi-styles "^3.2.1"
- escape-string-regexp "^1.0.5"
- supports-color "^5.3.0"
-
cheerio@^1.0.0-rc.1:
version "1.0.0-rc.2"
resolved "https://registry.yarnpkg.com/cheerio/-/cheerio-1.0.0-rc.2.tgz#4b9f53a81b27e4d5dac31c0ffd0cfa03cc6830db"
@@ -626,18 +706,6 @@ code-block-writer@9.4.1:
resolved "https://registry.yarnpkg.com/code-block-writer/-/code-block-writer-9.4.1.tgz#1448fca79dfc7a3649000f4c85be6bc770604c4c"
integrity sha512-LHAB+DL4YZDcwK8y/kAxZ0Lf/ncwLh/Ux4cTVWbPwIdrf1gPxXiPcwpz8r8/KqXu1aD+Raz46EOxDjFlbyO6bA==
-color-convert@^1.9.0:
- version "1.9.3"
- resolved "https://registry.yarnpkg.com/color-convert/-/color-convert-1.9.3.tgz#bb71850690e1f136567de629d2d5471deda4c1e8"
- integrity sha512-QfAUtd+vFdAtFQcC8CCyYt1fYWxSqAiK2cSD6zDB8N3cpsEBAvRxp9zOGg6G/SHHJYAT88/az/IuDGALsNVbGg==
- dependencies:
- color-name "1.1.3"
-
-color-name@1.1.3:
- version "1.1.3"
- resolved "https://registry.yarnpkg.com/color-name/-/color-name-1.1.3.tgz#a7d0558bd89c42f795dd42328f740831ca53bc25"
- integrity sha1-p9BVi9icQveV3UIyj3QIMcpTvCU=
-
color-support@^1.1.3:
version "1.1.3"
resolved "https://registry.yarnpkg.com/color-support/-/color-support-1.1.3.tgz#93834379a1cc9a0c61f82f52f0d04322251bd5a2"
@@ -672,16 +740,21 @@ command-line-args@^5.1.1:
lodash.camelcase "^4.3.0"
typical "^4.0.0"
-commander@^2.12.1, commander@^2.8.1:
- version "2.19.0"
- resolved "https://registry.yarnpkg.com/commander/-/commander-2.19.0.tgz#f6198aa84e5b83c46054b94ddedbfed5ee9ff12a"
- integrity sha512-6tvAOO+D6OENvRAh524Dh9jcfKTYDQAqvqezbCW82xj5X0pSrcpxtvRKHLG0yBY6SD7PSDrJaj+0AiOcKVd1Xg==
-
commander@^2.20.0, commander@~2.20.0:
version "2.20.0"
resolved "https://registry.yarnpkg.com/commander/-/commander-2.20.0.tgz#d58bb2b5c1ee8f87b0d340027e9e94e222c5a422"
integrity sha512-7j2y+40w61zy6YC2iRNpUe/NwhNyoXrYpHMrSunaMG64nRnaf96zO/KMQR4OyN/UnE5KLyEBnKHd4aG3rskjpQ==
+commander@^2.8.1:
+ version "2.19.0"
+ resolved "https://registry.yarnpkg.com/commander/-/commander-2.19.0.tgz#f6198aa84e5b83c46054b94ddedbfed5ee9ff12a"
+ integrity sha512-6tvAOO+D6OENvRAh524Dh9jcfKTYDQAqvqezbCW82xj5X0pSrcpxtvRKHLG0yBY6SD7PSDrJaj+0AiOcKVd1Xg==
+
+compare-version@^0.1.2:
+ version "0.1.2"
+ resolved "https://registry.yarnpkg.com/compare-version/-/compare-version-0.1.2.tgz#0162ec2d9351f5ddd59a9202cba935366a725080"
+ integrity sha1-AWLsLZNR9d3VmpICy6k1NmpyUIA=
+
concat-map@0.0.1:
version "0.0.1"
resolved "https://registry.yarnpkg.com/concat-map/-/concat-map-0.0.1.tgz#d8a96bd77fd68df7793a73036a3ba0d5405d477b"
@@ -770,14 +843,14 @@ debug-fabulous@0.0.X:
lazy-debug-legacy "0.0.X"
object-assign "4.1.0"
-debug@2.X:
+debug@2.X, debug@^2.6.8:
version "2.6.9"
resolved "https://registry.yarnpkg.com/debug/-/debug-2.6.9.tgz#5d128515df134ff327e90a4c93f4e077a536341f"
integrity sha512-bC7ElrdJaJnPbAP+1EotYvqZsb3ecl5wi6Bfi6BJTUcNowp6cvspg0jXznRTKDjm/E7AdgFBVeAPVMNcKGsHMA==
dependencies:
ms "2.0.0"
-debug@^4.1.1:
+debug@4, debug@^4.1.1:
version "4.1.1"
resolved "https://registry.yarnpkg.com/debug/-/debug-4.1.1.tgz#3b72260255109c6b589cee050f1d516139664791"
integrity sha512-pYAIzeRo8J6KPEaJ0VWOh5Pzkbw/RetuzehGM7QRRX5he4fPHx2rdKMB256ehJCkX+XRQm16eZLqLNS8RSZXZw==
@@ -816,11 +889,6 @@ diagnostic-channel@0.2.0:
dependencies:
semver "^5.3.0"
-diff@^3.2.0:
- version "3.5.0"
- resolved "https://registry.yarnpkg.com/diff/-/diff-3.5.0.tgz#800c0dd1e0a8bfbc95835c202ad220fe317e5a12"
- integrity sha512-A46qtFgd+g7pDZinpnwiRJtxbC1hpgf0uzP3iG89scHk0AUC7A1TGxf5OiiOUv/JMZR8GOt8hL900hV0bOy5xA==
-
dir-glob@^3.0.1:
version "3.0.1"
resolved "https://registry.yarnpkg.com/dir-glob/-/dir-glob-3.0.1.tgz#56dbf73d992a4a93ba1584f4534063fd2e41717f"
@@ -888,6 +956,18 @@ ecc-jsbn@~0.1.1:
dependencies:
jsbn "~0.1.0"
+electron-osx-sign@^0.4.16:
+ version "0.4.16"
+ resolved "https://registry.yarnpkg.com/electron-osx-sign/-/electron-osx-sign-0.4.16.tgz#0be8e579b2d9fa4c12d2a21f063898294b3434aa"
+ integrity sha512-ziMWfc3NmQlwnWLW6EaZq8nH2BWVng/atX5GWsGwhexJYpdW6hsg//MkAfRTRx1kR3Veiqkeiog1ibkbA4x0rg==
+ dependencies:
+ bluebird "^3.5.0"
+ compare-version "^0.1.2"
+ debug "^2.6.8"
+ isbinaryfile "^3.0.2"
+ minimist "^1.2.0"
+ plist "^3.0.1"
+
end-of-stream@^1.1.0:
version "1.4.4"
resolved "https://registry.yarnpkg.com/end-of-stream/-/end-of-stream-1.4.4.tgz#5ae64a5f45057baf3626ec14da0ca5e4b2431eb0"
@@ -900,20 +980,35 @@ entities@^1.1.1, entities@~1.1.1:
resolved "https://registry.yarnpkg.com/entities/-/entities-1.1.2.tgz#bdfa735299664dfafd34529ed4f8522a275fea56"
integrity sha512-f2LZMYl1Fzu7YSBKg+RoROelpOaNrcGmE9AZubeDfrCEia483oW4MI4VyFd5VNHIgQ/7qm1I0wUHK1eJnn2y2w==
-escape-string-regexp@^1.0.2, escape-string-regexp@^1.0.5:
+escape-string-regexp@^1.0.2:
version "1.0.5"
resolved "https://registry.yarnpkg.com/escape-string-regexp/-/escape-string-regexp-1.0.5.tgz#1b61c0562190a8dff6ae3bb2cf0200ca130b86d4"
integrity sha1-G2HAViGQqN/2rjuyzwIAyhMLhtQ=
-esprima@^4.0.0:
- version "4.0.1"
- resolved "https://registry.yarnpkg.com/esprima/-/esprima-4.0.1.tgz#13b04cdb3e6c5d19df91ab6987a8695619b0aa71"
- integrity sha512-eGuFFw7Upda+g4p+QHvnW0RyTX/SVeJBDM/gCtMARO0cLuT2HcEKnTPvhjV6aGeqrCB/sbNop0Kszm0jsaWU4A==
+eslint-scope@^5.0.0:
+ version "5.0.0"
+ resolved "https://registry.yarnpkg.com/eslint-scope/-/eslint-scope-5.0.0.tgz#e87c8887c73e8d1ec84f1ca591645c358bfc8fb9"
+ integrity sha512-oYrhJW7S0bxAFDvWqzvMPRm6pcgcnWc4QnofCAqRTRfQC0JcwenzGglTtsLyIuuWFfkqDG9vz67cnttSd53djw==
+ dependencies:
+ esrecurse "^4.1.0"
+ estraverse "^4.1.1"
-esutils@^2.0.2:
- version "2.0.2"
- resolved "https://registry.yarnpkg.com/esutils/-/esutils-2.0.2.tgz#0abf4f1caa5bcb1f7a9d8acc6dea4faaa04bac9b"
- integrity sha1-Cr9PHKpbyx96nYrMbepPqqBLrJs=
+eslint-visitor-keys@^1.1.0:
+ version "1.1.0"
+ resolved "https://registry.yarnpkg.com/eslint-visitor-keys/-/eslint-visitor-keys-1.1.0.tgz#e2a82cea84ff246ad6fb57f9bde5b46621459ec2"
+ integrity sha512-8y9YjtM1JBJU/A9Kc+SbaOV4y29sSWckBwMHa+FGtVj5gN/sbnKDf6xJUl+8g7FAij9LVaP8C24DUiH/f/2Z9A==
+
+esrecurse@^4.1.0:
+ version "4.2.1"
+ resolved "https://registry.yarnpkg.com/esrecurse/-/esrecurse-4.2.1.tgz#007a3b9fdbc2b3bb87e4879ea19c92fdbd3942cf"
+ integrity sha512-64RBB++fIOAXPw3P9cy89qfMlvZEXZkqqJkjqqXIvzP5ezRZjW+lPWjw35UX/3EhUPFYbg5ER4JYgDw4007/DQ==
+ dependencies:
+ estraverse "^4.1.0"
+
+estraverse@^4.1.0, estraverse@^4.1.1:
+ version "4.3.0"
+ resolved "https://registry.yarnpkg.com/estraverse/-/estraverse-4.3.0.tgz#398ad3f3c5a24948be7725e83d11a7de28cdbd1d"
+ integrity sha512-39nnKffWz8xN1BU/2c79n9nB9HDzo0niYUqx6xyqUnyoAnQyyWpOTdZEeiCch8BBu515t4wp9ZmgVfVhn9EBpw==
execa@^1.0.0:
version "1.0.0"
@@ -1078,7 +1173,7 @@ glob-parent@^5.0.0:
dependencies:
is-glob "^4.0.1"
-glob@^7.0.6, glob@^7.1.1:
+glob@^7.0.6:
version "7.1.3"
resolved "https://registry.yarnpkg.com/glob/-/glob-7.1.3.tgz#3960832d3f1574108342dafd3a67b332c0969df1"
integrity sha512-vcfuiIxogLV4DlGBHIUOwI0IbrJ8HWPc4MU7HzviGeNho/UJDfi6B5p3sHeWIQ0KGIU0Jpxi5ZHxemQfLkkAwQ==
@@ -1102,6 +1197,18 @@ glob@^7.1.3:
once "^1.3.0"
path-is-absolute "^1.0.0"
+glob@^7.1.6:
+ version "7.1.6"
+ resolved "https://registry.yarnpkg.com/glob/-/glob-7.1.6.tgz#141f33b81a7c2492e125594307480c46679278a6"
+ integrity sha512-LwaxwyZ72Lk7vZINtNNrywX0ZuLyStrdDtabefZKAY5ZGJhVtgdznluResxNmPitE0SAO+O26sWTHeKSI2wMBA==
+ dependencies:
+ fs.realpath "^1.0.0"
+ inflight "^1.0.4"
+ inherits "2"
+ minimatch "^3.0.4"
+ once "^1.3.0"
+ path-is-absolute "^1.0.0"
+
globby@^10.0.1:
version "10.0.1"
resolved "https://registry.yarnpkg.com/globby/-/globby-10.0.1.tgz#4782c34cb75dd683351335c5829cc3420e606b22"
@@ -1238,11 +1345,6 @@ has-ansi@^2.0.0:
dependencies:
ansi-regex "^2.0.0"
-has-flag@^3.0.0:
- version "3.0.0"
- resolved "https://registry.yarnpkg.com/has-flag/-/has-flag-3.0.0.tgz#b5d454dc2199ae225699f3467e5a07f3b955bafd"
- integrity sha1-tdRU3CGZriJWmfNGfloH87lVuv0=
-
has-gulplog@^0.1.0:
version "0.1.0"
resolved "https://registry.yarnpkg.com/has-gulplog/-/has-gulplog-0.1.0.tgz#6414c82913697da51590397dafb12f22967811ce"
@@ -1318,12 +1420,18 @@ http-signature@~1.2.0:
jsprim "^1.2.2"
sshpk "^1.7.0"
-iconv-lite@0.4.23:
- version "0.4.23"
- resolved "https://registry.yarnpkg.com/iconv-lite/-/iconv-lite-0.4.23.tgz#297871f63be507adcfbfca715d0cd0eed84e9a63"
- integrity sha512-neyTUVFtahjf0mB3dZT77u+8O0QB89jFdnBkd5P1JgYPbPaia3gXXOVL2fq8VyU2gMMD7SaN7QukTB/pmXYvDA==
+https-proxy-agent@^4.0.0:
+ version "4.0.0"
+ resolved "https://registry.yarnpkg.com/https-proxy-agent/-/https-proxy-agent-4.0.0.tgz#702b71fb5520a132a66de1f67541d9e62154d82b"
+ integrity sha512-zoDhWrkR3of1l9QAL8/scJZyLu8j/gBkcwcaQOZh7Gyh/+uJQzGVETdgT30akuwkpL8HTRfssqI3BZuV18teDg==
dependencies:
- safer-buffer ">= 2.1.2 < 3"
+ agent-base "5"
+ debug "4"
+
+iconv-lite-umd@0.6.8:
+ version "0.6.8"
+ resolved "https://registry.yarnpkg.com/iconv-lite-umd/-/iconv-lite-umd-0.6.8.tgz#5ad310ec126b260621471a2d586f7f37b9958ec0"
+ integrity sha512-zvXJ5gSwMC9JD3wDzH8CoZGc1pbiJn12Tqjk8BXYCnYz3hYL5GRjHW8LEykjXhV9WgNGI4rgpgHcbIiBfrRq6A==
ignore@^5.1.1:
version "5.1.2"
@@ -1436,6 +1544,13 @@ isarray@~1.0.0:
resolved "https://registry.yarnpkg.com/isarray/-/isarray-1.0.0.tgz#bb935d48582cba168c06834957a54a3e07124f11"
integrity sha1-u5NdSFgsuhaMBoNJV6VKPgcSTxE=
+isbinaryfile@^3.0.2:
+ version "3.0.3"
+ resolved "https://registry.yarnpkg.com/isbinaryfile/-/isbinaryfile-3.0.3.tgz#5d6def3edebf6e8ca8cae9c30183a804b5f8be80"
+ integrity sha512-8cJBL5tTd2OS0dM4jz07wQd5g0dCCqIhUxPIGtZfa5L6hWlvV5MHTITy/DBAsF+Oe2LS1X3krBUhNwaGUWpWxw==
+ dependencies:
+ buffer-alloc "^1.2.0"
+
isexe@^2.0.0:
version "2.0.0"
resolved "https://registry.yarnpkg.com/isexe/-/isexe-2.0.0.tgz#e8fbf374dc556ff8947a10dcb0572d633f2cfa10"
@@ -1451,19 +1566,6 @@ isstream@~0.1.2:
resolved "https://registry.yarnpkg.com/isstream/-/isstream-0.1.2.tgz#47e63f7af55afa6f92e1500e690eb8b8529c099a"
integrity sha1-R+Y/evVa+m+S4VAOaQ64uFKcCZo=
-js-tokens@^3.0.2:
- version "3.0.2"
- resolved "https://registry.yarnpkg.com/js-tokens/-/js-tokens-3.0.2.tgz#9866df395102130e38f7f996bceb65443209c25b"
- integrity sha1-mGbfOVECEw449/mWvOtlRDIJwls=
-
-js-yaml@^3.7.0:
- version "3.12.0"
- resolved "https://registry.yarnpkg.com/js-yaml/-/js-yaml-3.12.0.tgz#eaed656ec8344f10f527c6bfa1b6e2244de167d1"
- integrity sha512-PIt2cnwmPfL4hKNwqeiuz4bKfnzHTBv6HyVgjahA6mPLwPDzjDWrplJBMjHUFxku/N3FlmrbyPclad+I+4mJ3A==
- dependencies:
- argparse "^1.0.7"
- esprima "^4.0.0"
-
jsbn@~0.1.0:
version "0.1.1"
resolved "https://registry.yarnpkg.com/jsbn/-/jsbn-0.1.1.tgz#a5e654c2e5a2deb5f201d96cefbca80c0ef2f513"
@@ -1498,6 +1600,11 @@ json-stringify-safe@~5.0.1:
resolved "https://registry.yarnpkg.com/json-stringify-safe/-/json-stringify-safe-5.0.1.tgz#1296a2d58fd45f19a0f6ce01d65701e2c735b6eb"
integrity sha1-Epai1Y/UXxmg9s4B1lcB4sc1tus=
+jsonc-parser@^2.3.0:
+ version "2.3.0"
+ resolved "https://registry.yarnpkg.com/jsonc-parser/-/jsonc-parser-2.3.0.tgz#7c7fc988ee1486d35734faaaa866fadb00fa91ee"
+ integrity sha512-b0EBt8SWFNnixVdvoR2ZtEGa9ZqLhbJnOjezn+WP+8kspFm+PFYDN8Z4Bc7pRlDjvuVcADSUkroIuTWWn/YiIA==
+
jsonfile@^4.0.0:
version "4.0.0"
resolved "https://registry.yarnpkg.com/jsonfile/-/jsonfile-4.0.0.tgz#8771aae0799b64076b76640fca058f9c10e33ecb"
@@ -1641,10 +1748,15 @@ lodash.templatesettings@^3.0.0:
lodash._reinterpolate "^3.0.0"
lodash.escape "^3.0.0"
+lodash.unescape@4.0.1:
+ version "4.0.1"
+ resolved "https://registry.yarnpkg.com/lodash.unescape/-/lodash.unescape-4.0.1.tgz#bf2249886ce514cda112fae9218cdc065211fc9c"
+ integrity sha1-vyJJiGzlFM2hEvrpIYzcBlIR/Jw=
+
lodash@^4.15.0, lodash@^4.17.10:
- version "4.17.11"
- resolved "https://registry.yarnpkg.com/lodash/-/lodash-4.17.11.tgz#b39ea6229ef607ecd89e2c8df12536891cac9b8d"
- integrity sha512-cQKh8igo5QUhZ7lg38DYWAxMvjSAKG0A8wGSVimP07SIUEK2UO+arSRKbRZWtelMtN5V0Hkwh5ryOto/SshYIg==
+ version "4.17.19"
+ resolved "https://registry.yarnpkg.com/lodash/-/lodash-4.17.19.tgz#e48ddedbe30b3321783c5b4301fbd353bc1e4a4b"
+ integrity sha512-JNvd8XER9GQX0v2qJgsaN/mzFCNA5BRe/j8JN9d+tWyGLSodKQHKFicdwNYzWwI3wjRnaKPsGj1XkBjx/F96DQ==
macos-release@^2.2.0:
version "2.3.0"
@@ -1736,10 +1848,10 @@ minimatch@3.0.4, minimatch@^3.0.3, minimatch@^3.0.4:
dependencies:
brace-expansion "^1.1.7"
-minimist@^1.1.0, minimist@^1.2.0:
- version "1.2.0"
- resolved "https://registry.yarnpkg.com/minimist/-/minimist-1.2.0.tgz#a35008b20f41383eec1fb914f4cd5df79a264284"
- integrity sha1-o1AIsg9BOD7sH7kU9M1d95omQoQ=
+minimist@^1.1.0, minimist@^1.2.0, minimist@^1.2.3:
+ version "1.2.3"
+ resolved "https://registry.yarnpkg.com/minimist/-/minimist-1.2.3.tgz#3db5c0765545ab8637be71f333a104a965a9ca3f"
+ integrity sha512-+bMdgqjMN/Z77a6NlY/I3U5LlRDbnmaAk6lDveAPKwSpcPM4tKAuYsvYF8xjhOPXhOYGe/73vVLVez5PW+jqhw==
minimist@~0.0.1:
version "0.0.10"
@@ -1900,11 +2012,6 @@ path-key@^2.0.0, path-key@^2.0.1:
resolved "https://registry.yarnpkg.com/path-key/-/path-key-2.0.1.tgz#411cadb574c5a140d3a4b1910d40d80cc9f40b40"
integrity sha1-QRyttXTFoUDTpLGRDUDYDMn0C0A=
-path-parse@^1.0.5:
- version "1.0.6"
- resolved "https://registry.yarnpkg.com/path-parse/-/path-parse-1.0.6.tgz#d62dbb5679405d72c4737ec58600e9ddcf06d24c"
- integrity sha512-GSmOT2EbHrINBf9SR7CDELwlJ8AENk3Qn7OikK4nFYAu3Ote2+JYNVvkpAEQm3/TLNEJFD/xZJjzyxg3KBWOzw==
-
path-type@^4.0.0:
version "4.0.0"
resolved "https://registry.yarnpkg.com/path-type/-/path-type-4.0.0.tgz#84ed01c0a7ba380afe09d90a8c180dcd9d03043b"
@@ -1930,6 +2037,15 @@ picomatch@^2.0.5:
resolved "https://registry.yarnpkg.com/picomatch/-/picomatch-2.0.7.tgz#514169d8c7cd0bdbeecc8a2609e34a7163de69f6"
integrity sha512-oLHIdio3tZ0qH76NybpeneBhYVj0QFTfXEFTc/B3zKQspYfYYkWYgFsmzo+4kvId/bQRcNkVeguI3y+CD22BtA==
+plist@^3.0.1:
+ version "3.0.1"
+ resolved "https://registry.yarnpkg.com/plist/-/plist-3.0.1.tgz#a9b931d17c304e8912ef0ba3bdd6182baf2e1f8c"
+ integrity sha512-GpgvHHocGRyQm74b6FWEZZVRroHKE1I0/BTjAmySaohK+cUn+hZpbqXkc3KWgW3gQYkqcQej35FohcT0FRlkRQ==
+ dependencies:
+ base64-js "^1.2.3"
+ xmlbuilder "^9.0.7"
+ xmldom "0.1.x"
+
prettyjson@1.2.1:
version "1.2.1"
resolved "https://registry.yarnpkg.com/prettyjson/-/prettyjson-1.2.1.tgz#fcffab41d19cab4dfae5e575e64246619b12d289"
@@ -1953,6 +2069,11 @@ process-nextick-args@~2.0.0:
resolved "https://registry.yarnpkg.com/process-nextick-args/-/process-nextick-args-2.0.0.tgz#a37d732f4271b4ab1ad070d35508e8290788ffaa"
integrity sha512-MtEC1TqN0EU5nephaJ4rAtThHtC86dNN9qCuEhtshvpVBkAW5ZO7BASN9REnF9eoXGcRub+pFuKEpOHE+HbEMw==
+proxy-from-env@^1.1.0:
+ version "1.1.0"
+ resolved "https://registry.yarnpkg.com/proxy-from-env/-/proxy-from-env-1.1.0.tgz#e102f16ca355424865755d2c9e8ea4f24d58c3e2"
+ integrity sha512-D+zkORCbA9f1tdWRK0RaCR3GPv50cMxcrz4X8k5LTSUD1Dkw47mKJEZQNunItRTkWwgtaUSo1RVFRIG9ZXiFYg==
+
pump@^3.0.0:
version "3.0.0"
resolved "https://registry.yarnpkg.com/pump/-/pump-3.0.0.tgz#b4a2116815bde2f4e1ea602354e8c75565107a64"
@@ -2103,13 +2224,6 @@ resolve-url@^0.2.1:
resolved "https://registry.yarnpkg.com/resolve-url/-/resolve-url-0.2.1.tgz#2c637fe77c893afd2a663fe21aa9080068e2052a"
integrity sha1-LGN/53yJOv0qZj/iGqkIAGjiBSo=
-resolve@^1.3.2:
- version "1.8.1"
- resolved "https://registry.yarnpkg.com/resolve/-/resolve-1.8.1.tgz#82f1ec19a423ac1fbd080b0bab06ba36e84a7a26"
- integrity sha512-AicPrAC7Qu1JxPCZ9ZgCZlY35QgFnNqc+0LtbRNxnVw4TXvjQ72wnuL9JQcEBgXkI9JM8MsT9kaQoHcpCRJOYA==
- dependencies:
- path-parse "^1.0.5"
-
reusify@^1.0.0:
version "1.0.4"
resolved "https://registry.yarnpkg.com/reusify/-/reusify-1.0.4.tgz#90da382b1e126efc02146e90845a88db12925d76"
@@ -2135,11 +2249,6 @@ safe-buffer@~5.1.0, safe-buffer@~5.1.1:
resolved "https://registry.yarnpkg.com/safe-buffer/-/safe-buffer-5.1.2.tgz#991ec69d296e0313747d59bdfd2b745c35f8828d"
integrity sha512-Gd2UZBJDkXlY7GbJxfsE8/nvKkUEU1G38c1siN6QP6a9PT9MmHB8GnpscSmMJSoF8LOIrt8ud/wPtojys4G6+g==
-"safer-buffer@>= 2.1.2 < 3":
- version "2.1.2"
- resolved "https://registry.yarnpkg.com/safer-buffer/-/safer-buffer-2.1.2.tgz#44fa161b0187b9549dd84bb91802f9bd8385cd6a"
- integrity sha512-YZo3K82SD7Riyi0E1EQPojLz7kpepnSQI9IyPbHHg1XXXevb5dJI7tpyN2ADxGcQbHG7vcyRHk0cbwqcQriUtg==
-
sax@0.5.2:
version "0.5.2"
resolved "https://registry.yarnpkg.com/sax/-/sax-0.5.2.tgz#735ffaa39a1cff8ffb9598f0223abdb03a9fb2ea"
@@ -2165,6 +2274,11 @@ semver@^5.5.0:
resolved "https://registry.yarnpkg.com/semver/-/semver-5.7.1.tgz#a954f931aeba508d307bbf069eff0c01c96116f7"
integrity sha512-sauaDf/PZdVgrLTNYHRtpXa1iRiKcaebiKQ1BJdpQlWH2lCvexQdX55snPFyK7QzpudqbCI0qXFfOasHdyNDGQ==
+semver@^6.3.0:
+ version "6.3.0"
+ resolved "https://registry.yarnpkg.com/semver/-/semver-6.3.0.tgz#ee0a64c8af5e8ceea67687b133761e1becbd1d3d"
+ integrity sha512-b39TBaTSfV6yBrapU89p5fKekE2m/NwnDocOVruQFS1/veMgdzuPcnOM34M6CwxW8jH/lxEa5rBoDeUwu5HHTw==
+
shebang-command@^1.2.0:
version "1.2.0"
resolved "https://registry.yarnpkg.com/shebang-command/-/shebang-command-1.2.0.tgz#44aac65b695b03398968c39f363fee5deafdf1ea"
@@ -2301,13 +2415,6 @@ supports-color@^2.0.0:
resolved "https://registry.yarnpkg.com/supports-color/-/supports-color-2.0.0.tgz#535d045ce6b6363fa40117084629995e9df324c7"
integrity sha1-U10EXOa2Nj+kARcIRimZXp3zJMc=
-supports-color@^5.3.0:
- version "5.5.0"
- resolved "https://registry.yarnpkg.com/supports-color/-/supports-color-5.5.0.tgz#e2e69a44ac8772f78a1ec0b35b689df6530efc8f"
- integrity sha512-QjVjwdXIt408MIiAqCX4oUKsgU2EqAGzs2Ppkm4aQYbjm+ZEWEcW4SfFNTr4uMNZma0ey4f5lgLrkB0aX0QMow==
- dependencies:
- has-flag "^3.0.0"
-
terser@*:
version "4.2.1"
resolved "https://registry.yarnpkg.com/terser/-/terser-4.2.1.tgz#1052cfe17576c66e7bc70fcc7119f22b155bdac1"
@@ -2381,7 +2488,7 @@ ts-morph@^3.1.3:
multimatch "^4.0.0"
typescript "^3.0.1"
-tslib@^1.8.0, tslib@^1.8.1:
+tslib@^1.8.1:
version "1.9.3"
resolved "https://registry.yarnpkg.com/tslib/-/tslib-1.9.3.tgz#d7e4dd79245d85428c4d7e4822a79917954ca286"
integrity sha512-4krF8scpejhaOgqzBEcGM7yDIEfi0/8+8zDRZhNZZ2kjmHJ4hv3zCbQWxoJGz1iw5U0Jl0nma13xzHXcncMavQ==
@@ -2391,28 +2498,10 @@ tslib@^1.9.3:
resolved "https://registry.yarnpkg.com/tslib/-/tslib-1.10.0.tgz#c3c19f95973fb0a62973fb09d90d961ee43e5c8a"
integrity sha512-qOebF53frne81cf0S9B41ByenJ3/IuH8yJKngAX35CmiZySA0khhkovshKK+jGCaMnVomla7gVlIcc3EvKPbTQ==
-tslint@^5.9.1:
- version "5.11.0"
- resolved "https://registry.yarnpkg.com/tslint/-/tslint-5.11.0.tgz#98f30c02eae3cde7006201e4c33cb08b48581eed"
- integrity sha1-mPMMAurjzecAYgHkwzywi0hYHu0=
- dependencies:
- babel-code-frame "^6.22.0"
- builtin-modules "^1.1.1"
- chalk "^2.3.0"
- commander "^2.12.1"
- diff "^3.2.0"
- glob "^7.1.1"
- js-yaml "^3.7.0"
- minimatch "^3.0.4"
- resolve "^1.3.2"
- semver "^5.3.0"
- tslib "^1.8.0"
- tsutils "^2.27.2"
-
-tsutils@^2.27.2:
- version "2.29.0"
- resolved "https://registry.yarnpkg.com/tsutils/-/tsutils-2.29.0.tgz#32b488501467acbedd4b85498673a0812aca0b99"
- integrity sha512-g5JVHCIJwzfISaXpXE1qvNalca5Jwob6FjI4AoPlqMusJ6ftFE7IkkFoMhVLRgK+4Kx3gkzb8UZK5t5yTTvEmA==
+tsutils@^3.17.1:
+ version "3.17.1"
+ resolved "https://registry.yarnpkg.com/tsutils/-/tsutils-3.17.1.tgz#ed719917f11ca0dee586272b2ac49e015a2dd759"
+ integrity sha512-kzeQ5B8H3w60nFY2g8cJIuH7JDpsALXySGtwGJ0p2LSjLgay3NdIpqq5SoOBe46bKDW2iq25irHCr8wjomUS2g==
dependencies:
tslib "^1.8.1"
@@ -2441,16 +2530,16 @@ typed-rest-client@^0.9.0:
tunnel "0.0.4"
underscore "1.8.3"
-typescript@3.7.2:
- version "3.7.2"
- resolved "https://registry.yarnpkg.com/typescript/-/typescript-3.7.2.tgz#27e489b95fa5909445e9fef5ee48d81697ad18fb"
- integrity sha512-ml7V7JfiN2Xwvcer+XAf2csGO1bPBdRbFCkYBczNZggrBZ9c7G3riSUeJmqEU5uOtXNPMhE3n+R4FA/3YOAWOQ==
-
typescript@^3.0.1:
version "3.5.3"
resolved "https://registry.yarnpkg.com/typescript/-/typescript-3.5.3.tgz#c830f657f93f1ea846819e929092f5fe5983e977"
integrity sha512-ACzBtm/PhXBDId6a6sDJfroT2pOWt/oOnk4/dElG5G33ZL776N3Y6/6bKZJBFpd+b05F3Ct9qDjMeJmRWtE2/g==
+typescript@^4.1.0-dev.20200824:
+ version "4.1.0-dev.20200824"
+ resolved "https://registry.yarnpkg.com/typescript/-/typescript-4.1.0-dev.20200824.tgz#34c92d9b6e5124600658c0d4e9b8c125beaf577d"
+ integrity sha512-hTJfocmebnMKoqRw/xs3bL61z87XXtvOUwYtM7zaCX9mAvnfdo1x1bzQlLZAsvdzRIgAHPJQYbqYHKygWkDw6g==
+
typical@^4.0.0:
version "4.0.0"
resolved "https://registry.yarnpkg.com/typical/-/typical-4.0.0.tgz#cbeaff3b9d7ae1e2bbfaf5a4e6f11eccfde94fc4"
@@ -2581,19 +2670,22 @@ vsce@1.48.0:
yauzl "^2.3.1"
yazl "^2.2.2"
-vscode-ripgrep@^1.5.6:
- version "1.5.7"
- resolved "https://registry.yarnpkg.com/vscode-ripgrep/-/vscode-ripgrep-1.5.7.tgz#acb6b548af488a4bca5d0f1bb5faf761343289ce"
- integrity sha512-/Vsz/+k8kTvui0q3O74pif9FK0nKopgFTiGNVvxicZANxtSA8J8gUE9GQ/4dpi7D/2yI/YVORszwVskFbz46hQ==
+vscode-ripgrep@^1.6.2:
+ version "1.6.2"
+ resolved "https://registry.yarnpkg.com/vscode-ripgrep/-/vscode-ripgrep-1.6.2.tgz#fb912c7465699f10ce0218a6676cc632c77369b4"
+ integrity sha512-jkZEWnQFcE+QuQFfxQXWcWtDafTmgkp3DjMKawDkajZwgnDlGKpFp15ybKrZNVTi1SLEF/12BzxYSZVVZ2XrkA==
+ dependencies:
+ https-proxy-agent "^4.0.0"
+ proxy-from-env "^1.1.0"
-vscode-telemetry-extractor@^1.5.4:
- version "1.5.4"
- resolved "https://registry.yarnpkg.com/vscode-telemetry-extractor/-/vscode-telemetry-extractor-1.5.4.tgz#bcb0d17667fa1b77715e3a3bf372ade18f846782"
- integrity sha512-MN9LNPo0Rc6cy3sIWTAG97PTWkEKdRnP0VeYoS8vjKSNtG9CAsrUxHgFfYoHm2vNK/ijd0a4NzETyVGO2kT6hw==
+vscode-telemetry-extractor@^1.6.0:
+ version "1.6.0"
+ resolved "https://registry.yarnpkg.com/vscode-telemetry-extractor/-/vscode-telemetry-extractor-1.6.0.tgz#e9d9c1d24863cce8d3d715f0287de3b31eb90c56"
+ integrity sha512-zSxvkbyAMa1lTRGIHfGg7gW2e9Sey+2zGYD19uNWCsVEfoXAr2NB6uzb0sNHtbZ2SSqxSePmFXzBAavsudT5fw==
dependencies:
command-line-args "^5.1.1"
ts-morph "^3.1.3"
- vscode-ripgrep "^1.5.6"
+ vscode-ripgrep "^1.6.2"
vso-node-api@6.1.2-preview:
version "6.1.2-preview"
@@ -2649,11 +2741,21 @@ xmlbuilder@0.4.3:
resolved "https://registry.yarnpkg.com/xmlbuilder/-/xmlbuilder-0.4.3.tgz#c4614ba74e0ad196e609c9272cd9e1ddb28a8a58"
integrity sha1-xGFLp04K0ZbmCcknLNnh3bKKilg=
+xmlbuilder@^9.0.7:
+ version "9.0.7"
+ resolved "https://registry.yarnpkg.com/xmlbuilder/-/xmlbuilder-9.0.7.tgz#132ee63d2ec5565c557e20f4c22df9aca686b10d"
+ integrity sha1-Ey7mPS7FVlxVfiD0wi35rKaGsQ0=
+
xmlbuilder@~9.0.1:
version "9.0.4"
resolved "https://registry.yarnpkg.com/xmlbuilder/-/xmlbuilder-9.0.4.tgz#519cb4ca686d005a8420d3496f3f0caeecca580f"
integrity sha1-UZy0ymhtAFqEINNJbz8MruzKWA8=
+xmldom@0.1.x:
+ version "0.1.31"
+ resolved "https://registry.yarnpkg.com/xmldom/-/xmldom-0.1.31.tgz#b76c9a1bd9f0a9737e5a72dc37231cf38375e2ff"
+ integrity sha512-yS2uJflVQs6n+CyjHoaBmVSqIDevTAWrzMmjG1Gc7h1qQ7uVozNhEPJAwZXWyGQ/Gafo3fCwrcaokezLPupVyQ==
+
xtend@~4.0.1:
version "4.0.1"
resolved "https://registry.yarnpkg.com/xtend/-/xtend-4.0.1.tgz#a5c6d532be656e23db820efb943a1f04998d63af"
diff --git a/cglicenses.json b/cglicenses.json
index 1e9287cbaf28b..0da22bd9f5728 100644
--- a/cglicenses.json
+++ b/cglicenses.json
@@ -81,5 +81,283 @@
"prependLicenseText": [
"Copyright (c) Microsoft Corporation. All rights reserved."
]
+ },
+ {
+ // Reason: The license at https://github.com/rbuckton/reflect-metadata/blob/master/LICENSE
+ // does not include a clear Copyright statement (it's in https://github.com/rbuckton/reflect-metadata/blob/master/CopyrightNotice.txt).
+ "name": "reflect-metadata",
+ "prependLicenseText": [
+ "Copyright (c) Microsoft Corporation. All rights reserved."
+ ]
+ },
+ {
+ // Reason: The license at https://github.com/reem/rust-unreachable/blob/master/LICENSE-MIT
+ // cannot be found by the OSS tool automatically.
+ "name": "reem/rust-unreachable",
+ "fullLicenseText": [
+ "Copyright (c) 2015 The rust-unreachable Developers",
+ "",
+ "Permission is hereby granted, free of charge, to any person obtaining a copy",
+ "of this software and associated documentation files (the \"Software\"), to deal",
+ "in the Software without restriction, including without limitation the rights",
+ "to use, copy, modify, merge, publish, distribute, sublicense, and/or sell",
+ "copies of the Software, and to permit persons to whom the Software is",
+ "furnished to do so, subject to the following conditions:",
+ "",
+ "The above copyright notice and this permission notice shall be included in all",
+ "copies or substantial portions of the Software.",
+ "",
+ "THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR",
+ "IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,",
+ "FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE",
+ "AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER",
+ "LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,",
+ "OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE",
+ "SOFTWARE."
+ ]
+ },
+ {
+ // Reason: The license at https://github.com/reem/rust-void/blob/master/LICENSE-MIT
+ // cannot be found by the OSS tool automatically.
+ "name": "reem/rust-void",
+ "fullLicenseText": [
+ "Copyright (c) 2015 The rust-void Developers",
+ "",
+ "Permission is hereby granted, free of charge, to any person obtaining a copy",
+ "of this software and associated documentation files (the \"Software\"), to deal",
+ "in the Software without restriction, including without limitation the rights",
+ "to use, copy, modify, merge, publish, distribute, sublicense, and/or sell",
+ "copies of the Software, and to permit persons to whom the Software is",
+ "furnished to do so, subject to the following conditions:",
+ "",
+ "The above copyright notice and this permission notice shall be included in all",
+ "copies or substantial portions of the Software.",
+ "",
+ "THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR",
+ "IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,",
+ "FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE",
+ "AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER",
+ "LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,",
+ "OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE",
+ "SOFTWARE."
+ ]
+ },
+ {
+ // Reason: The license at https://github.com/mrhooray/crc-rs/blob/master/LICENSE-MIT
+ // cannot be found by the OSS tool automatically.
+ "name": "mrhooray/crc-rs",
+ "fullLicenseText": [
+ "MIT License",
+ "",
+ "Copyright (c) 2017 crc-rs Developers",
+ "",
+ "Permission is hereby granted, free of charge, to any person obtaining a copy",
+ "of this software and associated documentation files (the \"Software\"), to deal",
+ "in the Software without restriction, including without limitation the rights",
+ "to use, copy, modify, merge, publish, distribute, sublicense, and/or sell",
+ "copies of the Software, and to permit persons to whom the Software is",
+ "furnished to do so, subject to the following conditions:",
+ "",
+ "The above copyright notice and this permission notice shall be included in all",
+ "copies or substantial portions of the Software.",
+ "",
+ "THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR",
+ "IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,",
+ "FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE",
+ "AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER",
+ "LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,",
+ "OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE",
+ "SOFTWARE."
+ ]
+ },
+ {
+ // Reason: The license at https://github.com/floatdrop/pinkie/blob/master/license
+ // cannot be found by the OSS tool automatically.
+ "name": "pinkie",
+ "fullLicenseText": [
+ "The MIT License (MIT)",
+ "",
+ "Copyright (c) Vsevolod Strukchinsky (github.com/floatdrop)",
+ "",
+ "Permission is hereby granted, free of charge, to any person obtaining a copy",
+ "of this software and associated documentation files (the \"Software\"), to deal",
+ "in the Software without restriction, including without limitation the rights",
+ "to use, copy, modify, merge, publish, distribute, sublicense, and/or sell",
+ "copies of the Software, and to permit persons to whom the Software is",
+ "furnished to do so, subject to the following conditions:",
+ "",
+ "The above copyright notice and this permission notice shall be included in",
+ "all copies or substantial portions of the Software.",
+ "",
+ "THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR",
+ "IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,",
+ "FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE",
+ "AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER",
+ "LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,",
+ "OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN",
+ "THE SOFTWARE."
+ ]
+ },
+ {
+ "name": "big-integer",
+ "prependLicenseText": [
+ "Copyright released to public domain"
+ ]
+ },
+ {
+ // Reason: The license at https://github.com/justmoon/node-extend/blob/main/LICENSE
+ // cannot be found by the OSS tool automatically.
+ "name": "extend",
+ "fullLicenseText": [
+ "The MIT License (MIT)",
+ "",
+ "Copyright (c) 2014 Stefan Thomas",
+ "",
+ "Permission is hereby granted, free of charge, to any person obtaining",
+ "a copy of this software and associated documentation files (the",
+ "\"Software\"), to deal in the Software without restriction, including",
+ "without limitation the rights to use, copy, modify, merge, publish,",
+ "distribute, sublicense, and/or sell copies of the Software, and to",
+ "permit persons to whom the Software is furnished to do so, subject to",
+ "the following conditions:",
+ "",
+ "The above copyright notice and this permission notice shall be",
+ "included in all copies or substantial portions of the Software.",
+ "",
+ "THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND,",
+ "EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF",
+ "MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND",
+ "NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE",
+ "LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION",
+ "OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION",
+ "WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE."
+ ]
+ },
+ {
+ // Reason: The license at https://github.com/retep998/winapi-rs/blob/0.3/LICENSE-MIT
+ // cannot be found by the OSS tool automatically.
+ "name": "retep998/winapi-rs",
+ "fullLicenseText": [
+ "Copyright (c) 2015-2018 The winapi-rs Developers",
+ "",
+ "Permission is hereby granted, free of charge, to any person obtaining a copy",
+ "of this software and associated documentation files (the \"Software\"), to deal",
+ "in the Software without restriction, including without limitation the rights",
+ "to use, copy, modify, merge, publish, distribute, sublicense, and/or sell",
+ "copies of the Software, and to permit persons to whom the Software is",
+ "furnished to do so, subject to the following conditions:",
+ "",
+ "The above copyright notice and this permission notice shall be included in all",
+ "copies or substantial portions of the Software.",
+ "",
+ "THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR",
+ "IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,",
+ "FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE",
+ "AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER",
+ "LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,",
+ "OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE",
+ "SOFTWARE."
+ ]
+ },
+ {
+ // Reason: The license at https://github.com/digitaldesignlabs/es6-promisify/blob/main/LICENSE
+ // cannot be found by the OSS tool automatically.
+ "name": "es6-promisify",
+ "fullLicenseText": [
+ "Copyright (c) 2014 Mike Hall / Digital Design Labs",
+ "",
+ "Permission is hereby granted, free of charge, to any person obtaining a copy",
+ "of this software and associated documentation files (the \"Software\"), to deal",
+ "in the Software without restriction, including without limitation the rights",
+ "to use, copy, modify, merge, publish, distribute, sublicense, and/or sell",
+ "copies of the Software, and to permit persons to whom the Software is",
+ "furnished to do so, subject to the following conditions:",
+ "",
+ "The above copyright notice and this permission notice shall be included in all",
+ "copies or substantial portions of the Software.",
+ "",
+ "THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR",
+ "IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,",
+ "FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE",
+ "AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER",
+ "LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,",
+ "OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE",
+ "SOFTWARE."
+ ]
+ },
+ {
+ // Reason: The license at https://github.com/zkat/json-parse-better-errors/blob/latest/LICENSE.md
+ // cannot be found by the OSS tool automatically.
+ "name": "json-parse-better-errors",
+ "fullLicenseText": [
+ "Copyright 2017 Kat Marchán",
+ "",
+ "Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the",
+ "\"Software\"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute,",
+ "sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following",
+ "conditions:",
+ "",
+ "The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.",
+ "",
+ "THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE",
+ "WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS",
+ "OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR",
+ "OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE."
+ ]
+ },
+ {
+ // Reason: The license at https://github.com/time-rs/time/blob/main/LICENSE-MIT
+ // cannot be found by the OSS tool automatically.
+ "name": "time-rs/time",
+ "fullLicenseText": [
+ "Copyright (c) 2019 Jacob Pratt",
+ "",
+ "Permission is hereby granted, free of charge, to any person obtaining a copy",
+ "of this software and associated documentation files (the \"Software\"), to deal",
+ "in the Software without restriction, including without limitation the rights",
+ "to use, copy, modify, merge, publish, distribute, sublicense, and/or sell",
+ "copies of the Software, and to permit persons to whom the Software is",
+ "furnished to do so, subject to the following conditions:",
+ "",
+ "The above copyright notice and this permission notice shall be included in all",
+ "copies or substantial portions of the Software.",
+ "",
+ "THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR",
+ "IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,",
+ "FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE",
+ "AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER",
+ "LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,",
+ "OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE",
+ "SOFTWARE."
+ ]
+ },
+ {
+ // Reason: The license at https://github.com/colorjs/color-name/blob/master/LICENSE
+ // cannot be found by the OSS tool automatically.
+ "name": "color-name",
+ "fullLicenseText": [
+ "The MIT License (MIT)",
+ "Copyright (c) 2015 Dmitry Ivanov",
+ "",
+ "Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the \"Software\"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:",
+ "",
+ "The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.",
+ "",
+ "THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE."
+ ]
+ },
+ {
+ // Reason: The license cannot be found by the tool due to access controls on the repository
+ "name": "tas-client",
+ "fullLicenseText": [
+ "MIT License",
+ "Copyright (c) 2020 - present Microsoft Corporation",
+ "",
+ "Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the \"Software\"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:",
+ "",
+ "The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.",
+ "",
+ "THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE."
+ ]
}
]
diff --git a/cgmanifest.json b/cgmanifest.json
index 53dab5356a231..859af568b1bb2 100644
--- a/cgmanifest.json
+++ b/cgmanifest.json
@@ -6,7 +6,7 @@
"git": {
"name": "chromium",
"repositoryUrl": "https://chromium.googlesource.com/chromium/src",
- "commitHash": "91f08db83c2ce8c722ddf0911ead8f7c473bedfa"
+ "commitHash": "894fb9eb56c6cbda65e3c3ae9ada6d4cb5850cc9"
}
},
"licenseDetail": [
@@ -40,7 +40,7 @@
"SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE."
],
"isOnlyProductionDependency": true,
- "version": "76.0.3809.146"
+ "version": "83.0.4103.122"
},
{
"component": {
@@ -48,11 +48,11 @@
"git": {
"name": "nodejs",
"repositoryUrl": "https://github.com/nodejs/node",
- "commitHash": "64219741218aa87e259cf8257596073b8e747f0a"
+ "commitHash": "9622fed3fb2cffcea9efff6c8cb4cc2def99d75d"
}
},
"isOnlyProductionDependency": true,
- "version": "12.4.0"
+ "version": "12.14.1"
},
{
"component": {
@@ -60,12 +60,12 @@
"git": {
"name": "electron",
"repositoryUrl": "https://github.com/electron/electron",
- "commitHash": "dabaa7557a165cc107f89fd7c3e3c3210f9b5758"
+ "commitHash": "fb03807cd21915ddc3aa2521ba4f5ba14597bd7e"
}
},
"isOnlyProductionDependency": true,
"license": "MIT",
- "version": "6.1.2"
+ "version": "9.3.0"
},
{
"component": {
@@ -77,6 +77,40 @@
}
},
"isOnlyProductionDependency": true,
+ "licenseDetail": [
+ "Inno Setup License",
+ "==================",
+ "",
+ "Except where otherwise noted, all of the documentation and software included in the Inno Setup",
+ "package is copyrighted by Jordan Russell.",
+ "",
+ "Copyright (C) 1997-2020 Jordan Russell. All rights reserved.",
+ "Portions Copyright (C) 2000-2020 Martijn Laan. All rights reserved.",
+ "",
+ "This software is provided \"as-is,\" without any express or implied warranty. In no event shall the",
+ "author be held liable for any damages arising from the use of this software.",
+ "",
+ "Permission is granted to anyone to use this software for any purpose, including commercial",
+ "applications, and to alter and redistribute it, provided that the following conditions are met:",
+ "",
+ "1. All redistributions of source code files must retain all copyright notices that are currently in",
+ " place, and this list of conditions without modification.",
+ "",
+ "2. All redistributions in binary form must retain all occurrences of the above copyright notice and",
+ " web site addresses that are currently in place (for example, in the About boxes).",
+ "",
+ "3. The origin of this software must not be misrepresented; you must not claim that you wrote the",
+ " original software. If you use this software to distribute a product, an acknowledgment in the",
+ " product documentation would be appreciated but is not required.",
+ "",
+ "4. Modified versions in source or binary form must be plainly marked as such, and must not be",
+ " misrepresented as being the original software.",
+ "",
+ "",
+ "Jordan Russell",
+ "jr-2010 AT jrsoftware.org",
+ "https://jrsoftware.org/"
+ ],
"version": "5.5.6"
},
{
@@ -98,7 +132,7 @@
"git": {
"name": "vscode-codicons",
"repositoryUrl": "https://github.com/microsoft/vscode-codicons",
- "commitHash": "1f8534396cda86d3fa199b0e45ce110b55d794d0"
+ "commitHash": "f0caa623812a8ed5059516277675b4158d4c4867"
}
},
"license": "MIT and Creative Commons Attribution 4.0",
@@ -499,7 +533,7 @@
"git": {
"name": "ripgrep",
"repositoryUrl": "https://github.com/BurntSushi/ripgrep",
- "commitHash": "8a7db1a918e969b85cd933d8ed9fa5285b281ba4"
+ "commitHash": "973de50c9ef451da2cfcdfa86f2b2711d8d6ff48"
}
},
"isOnlyProductionDependency": true,
diff --git a/extensions/bat/language-configuration.json b/extensions/bat/language-configuration.json
index 2fb5445a34a47..e205fb5f5c4eb 100644
--- a/extensions/bat/language-configuration.json
+++ b/extensions/bat/language-configuration.json
@@ -1,6 +1,6 @@
{
"comments": {
- "lineComment": "REM"
+ "lineComment": "@REM"
},
"brackets": [
["{", "}"],
@@ -11,18 +11,19 @@
["{", "}"],
["[", "]"],
["(", ")"],
- ["\"", "\""]
+ { "open": "\"", "close": "\"", "notIn": ["string"] }
],
"surroundingPairs": [
["{", "}"],
["[", "]"],
["(", ")"],
+ ["%", "%"],
["\"", "\""]
],
"folding": {
"markers": {
- "start": "^\\s*(::\\s*|REM\\s+)#region",
- "end": "^\\s*(::\\s*|REM\\s+)#endregion"
+ "start": "^\\s*(::|REM|@REM)\\s*#region",
+ "end": "^\\s*(::|REM|@REM)\\s*#endregion"
}
}
}
diff --git a/extensions/bat/package.json b/extensions/bat/package.json
index 00bd84e4ae42f..dc543aef40272 100644
--- a/extensions/bat/package.json
+++ b/extensions/bat/package.json
@@ -23,7 +23,7 @@
}],
"snippets": [{
"language": "bat",
- "path": "./snippets/batchfile.snippets.json"
+ "path": "./snippets/batchfile.code-snippets"
}]
}
-}
\ No newline at end of file
+}
diff --git a/extensions/bat/snippets/batchfile.snippets.json b/extensions/bat/snippets/batchfile.code-snippets
similarity index 100%
rename from extensions/bat/snippets/batchfile.snippets.json
rename to extensions/bat/snippets/batchfile.code-snippets
diff --git a/extensions/bat/test/colorize-results/test_bat.json b/extensions/bat/test/colorize-results/test_bat.json
index 97155fafc8b8b..eb76b0e1d0419 100644
--- a/extensions/bat/test/colorize-results/test_bat.json
+++ b/extensions/bat/test/colorize-results/test_bat.json
@@ -488,7 +488,7 @@
"t": "source.batchfile constant.character.escape.batchfile",
"r": {
"dark_plus": "constant.character.escape: #D7BA7D",
- "light_plus": "constant.character.escape: #FF0000",
+ "light_plus": "constant.character.escape: #EE0000",
"dark_vs": "default: #D4D4D4",
"light_vs": "default: #000000",
"hc_black": "constant.character: #569CD6"
@@ -510,7 +510,7 @@
"t": "source.batchfile constant.character.escape.batchfile",
"r": {
"dark_plus": "constant.character.escape: #D7BA7D",
- "light_plus": "constant.character.escape: #FF0000",
+ "light_plus": "constant.character.escape: #EE0000",
"dark_vs": "default: #D4D4D4",
"light_vs": "default: #000000",
"hc_black": "constant.character: #569CD6"
diff --git a/extensions/clojure/language-configuration.json b/extensions/clojure/language-configuration.json
index 24f5248001560..a62cb968ece20 100644
--- a/extensions/clojure/language-configuration.json
+++ b/extensions/clojure/language-configuration.json
@@ -11,7 +11,7 @@
["{", "}"],
["[", "]"],
["(", ")"],
- ["\"", "\""]
+ { "open": "\"", "close": "\"", "notIn": ["string"] }
],
"surroundingPairs": [
["{", "}"],
@@ -22,4 +22,4 @@
"folding": {
"offSide": true
}
-}
\ No newline at end of file
+}
diff --git a/extensions/clojure/test/colorize-results/test_clj.json b/extensions/clojure/test/colorize-results/test_clj.json
index b7dd17d91e886..64b91983a1017 100644
--- a/extensions/clojure/test/colorize-results/test_clj.json
+++ b/extensions/clojure/test/colorize-results/test_clj.json
@@ -202,9 +202,9 @@
"t": "source.clojure meta.expression.clojure meta.definition.global.clojure constant.numeric.long.clojure",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -235,9 +235,9 @@
"t": "source.clojure meta.vector.clojure constant.numeric.long.clojure",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -257,9 +257,9 @@
"t": "source.clojure meta.vector.clojure constant.numeric.long.clojure",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -279,9 +279,9 @@
"t": "source.clojure meta.vector.clojure constant.numeric.long.clojure",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -345,9 +345,9 @@
"t": "source.clojure meta.vector.clojure constant.numeric.long.clojure",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -466,9 +466,9 @@
"t": "source.clojure meta.map.clojure constant.numeric.long.clojure",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -510,9 +510,9 @@
"t": "source.clojure meta.map.clojure constant.numeric.long.clojure",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -620,9 +620,9 @@
"t": "source.clojure meta.quoted-expression.clojure constant.numeric.long.clojure",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -642,9 +642,9 @@
"t": "source.clojure meta.quoted-expression.clojure constant.numeric.long.clojure",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -664,9 +664,9 @@
"t": "source.clojure meta.quoted-expression.clojure constant.numeric.long.clojure",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -1368,9 +1368,9 @@
"t": "source.clojure meta.quoted-expression.clojure constant.numeric.long.clojure",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -1390,9 +1390,9 @@
"t": "source.clojure meta.quoted-expression.clojure constant.numeric.long.clojure",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -1412,9 +1412,9 @@
"t": "source.clojure meta.quoted-expression.clojure constant.numeric.long.clojure",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -1522,9 +1522,9 @@
"t": "source.clojure meta.expression.clojure meta.vector.clojure constant.numeric.long.clojure",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -1566,9 +1566,9 @@
"t": "source.clojure meta.expression.clojure meta.vector.clojure constant.numeric.long.clojure",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -1610,9 +1610,9 @@
"t": "source.clojure meta.expression.clojure meta.vector.clojure constant.numeric.long.clojure",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -2567,9 +2567,9 @@
"t": "source.clojure meta.expression.clojure meta.definition.global.clojure meta.map.clojure constant.numeric.long.clojure",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -2611,9 +2611,9 @@
"t": "source.clojure meta.expression.clojure meta.definition.global.clojure meta.map.clojure constant.numeric.long.clojure",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -2721,9 +2721,9 @@
"t": "source.clojure meta.expression.clojure constant.numeric.long.clojure",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -3007,9 +3007,9 @@
"t": "source.clojure meta.expression.clojure meta.definition.global.clojure meta.expression.clojure meta.map.clojure constant.numeric.long.clojure",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -3321,4 +3321,4 @@
"hc_black": "comment: #7CA668"
}
}
-]
\ No newline at end of file
+]
diff --git a/extensions/coffeescript/language-configuration.json b/extensions/coffeescript/language-configuration.json
index fd4a1b88c87dd..c2b8662039d61 100644
--- a/extensions/coffeescript/language-configuration.json
+++ b/extensions/coffeescript/language-configuration.json
@@ -12,8 +12,8 @@
["{", "}"],
["[", "]"],
["(", ")"],
- ["\"", "\""],
- ["'", "'"]
+ { "open": "\"", "close": "\"", "notIn": ["string"] },
+ { "open": "'", "close": "'", "notIn": ["string"] }
],
"surroundingPairs": [
["{", "}"],
diff --git a/extensions/coffeescript/package.json b/extensions/coffeescript/package.json
index df5f8ff7c1f78..9bc96cca5d83b 100644
--- a/extensions/coffeescript/package.json
+++ b/extensions/coffeescript/package.json
@@ -28,7 +28,7 @@
],
"snippets": [{
"language": "coffeescript",
- "path": "./snippets/coffeescript.snippets.json"
+ "path": "./snippets/coffeescript.code-snippets"
}]
}
-}
\ No newline at end of file
+}
diff --git a/extensions/coffeescript/snippets/coffeescript.snippets.json b/extensions/coffeescript/snippets/coffeescript.code-snippets
similarity index 100%
rename from extensions/coffeescript/snippets/coffeescript.snippets.json
rename to extensions/coffeescript/snippets/coffeescript.code-snippets
diff --git a/extensions/coffeescript/test/colorize-results/test-regex_coffee.json b/extensions/coffeescript/test/colorize-results/test-regex_coffee.json
index 9daab0d553371..15f4b3cd38449 100644
--- a/extensions/coffeescript/test/colorize-results/test-regex_coffee.json
+++ b/extensions/coffeescript/test/colorize-results/test-regex_coffee.json
@@ -147,9 +147,9 @@
"t": "source.coffee constant.numeric.decimal.coffee",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -191,9 +191,9 @@
"t": "source.coffee constant.numeric.decimal.coffee",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -202,9 +202,9 @@
"t": "source.coffee constant.numeric.decimal.coffee",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -224,9 +224,9 @@
"t": "source.coffee constant.numeric.decimal.coffee",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -725,4 +725,4 @@
"hc_black": "string.regexp: #D16969"
}
}
-]
\ No newline at end of file
+]
diff --git a/extensions/coffeescript/test/colorize-results/test_coffee.json b/extensions/coffeescript/test/colorize-results/test_coffee.json
index d3de07d3f82de..9647307f073bd 100644
--- a/extensions/coffeescript/test/colorize-results/test_coffee.json
+++ b/extensions/coffeescript/test/colorize-results/test_coffee.json
@@ -1016,9 +1016,9 @@
"t": "source.coffee constant.numeric.decimal.coffee",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -1038,9 +1038,9 @@
"t": "source.coffee constant.numeric.decimal.coffee",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -1555,7 +1555,7 @@
"t": "source.coffee string.regexp.multiline.coffee keyword.control.anchor.regexp",
"r": {
"dark_plus": "keyword.control.anchor.regexp: #DCDCAA",
- "light_plus": "keyword.control.anchor.regexp: #FF0000",
+ "light_plus": "keyword.control.anchor.regexp: #EE0000",
"dark_vs": "keyword.control: #569CD6",
"light_vs": "keyword.control: #0000FF",
"hc_black": "keyword.control: #C586C0"
diff --git a/extensions/configuration-editing/.vscodeignore b/extensions/configuration-editing/.vscodeignore
index f12c396fd04d5..de8e6dc591301 100644
--- a/extensions/configuration-editing/.vscodeignore
+++ b/extensions/configuration-editing/.vscodeignore
@@ -3,4 +3,5 @@ src/**
tsconfig.json
out/**
extension.webpack.config.js
+extension-browser.webpack.config.js
yarn.lock
diff --git a/extensions/configuration-editing/extension-browser.webpack.config.js b/extensions/configuration-editing/extension-browser.webpack.config.js
new file mode 100644
index 0000000000000..de9416253737d
--- /dev/null
+++ b/extensions/configuration-editing/extension-browser.webpack.config.js
@@ -0,0 +1,21 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+//@ts-check
+
+'use strict';
+
+const withBrowserDefaults = require('../shared.webpack.config').browser;
+
+module.exports = withBrowserDefaults({
+ context: __dirname,
+ entry: {
+ extension: './src/configurationEditingMain.ts'
+ },
+ output: {
+ filename: 'configurationEditingMain.js'
+ }
+});
+
diff --git a/extensions/configuration-editing/extension.webpack.config.js b/extensions/configuration-editing/extension.webpack.config.js
index b474e65cbb130..1b18dd86a9911 100644
--- a/extensions/configuration-editing/extension.webpack.config.js
+++ b/extensions/configuration-editing/extension.webpack.config.js
@@ -12,7 +12,10 @@ const withDefaults = require('../shared.webpack.config');
module.exports = withDefaults({
context: __dirname,
entry: {
- extension: './src/extension.ts',
+ extension: './src/configurationEditingMain.ts',
+ },
+ output: {
+ filename: 'configurationEditingMain.js'
},
resolve: {
mainFields: ['module', 'main']
diff --git a/extensions/configuration-editing/package.json b/extensions/configuration-editing/package.json
index 5defc86cf5d9e..3afe9fa4332ab 100644
--- a/extensions/configuration-editing/package.json
+++ b/extensions/configuration-editing/package.json
@@ -12,14 +12,15 @@
"onLanguage:json",
"onLanguage:jsonc"
],
- "main": "./out/extension",
+ "main": "./out/configurationEditingMain",
+ "browser": "./dist/browser/configurationEditingMain",
"scripts": {
"compile": "gulp compile-extension:configuration-editing",
"watch": "gulp watch-extension:configuration-editing"
},
"dependencies": {
- "jsonc-parser": "^2.1.1",
- "vscode-nls": "^4.0.0"
+ "jsonc-parser": "^2.2.1",
+ "vscode-nls": "^4.1.1"
},
"contributes": {
"languages": [
@@ -53,7 +54,7 @@
"url": "vscode://schemas/keybindings"
},
{
- "fileMatch": "vscode://defaultsettings/*/*.json",
+ "fileMatch": "vscode://defaultsettings/*.json",
"url": "vscode://schemas/settings/default"
},
{
@@ -88,10 +89,18 @@
"fileMatch": "/.vscode/tasks.json",
"url": "vscode://schemas/tasks"
},
+ {
+ "fileMatch": "%APP_SETTINGS_HOME%/tasks.json",
+ "url": "vscode://schemas/tasks"
+ },
{
"fileMatch": "%APP_SETTINGS_HOME%/snippets/*.json",
"url": "vscode://schemas/snippets"
},
+ {
+ "fileMatch": "%APP_SETTINGS_HOME%/sync/snippets/preview/*.json",
+ "url": "vscode://schemas/snippets"
+ },
{
"fileMatch": "**/*.code-snippets",
"url": "vscode://schemas/global-snippets"
@@ -108,6 +117,10 @@
"fileMatch": "/.devcontainer.json",
"url": "./schemas/devContainer.schema.json"
},
+ {
+ "fileMatch": "%APP_SETTINGS_HOME%/globalStorage/ms-vscode-remote.remote-containers/nameConfigs/*.json",
+ "url": "./schemas/attachContainer.schema.json"
+ },
{
"fileMatch": "%APP_SETTINGS_HOME%/globalStorage/ms-vscode-remote.remote-containers/imageConfigs/*.json",
"url": "./schemas/attachContainer.schema.json"
diff --git a/extensions/configuration-editing/schemas/attachContainer.schema.json b/extensions/configuration-editing/schemas/attachContainer.schema.json
index 5f3d28abb89ec..2b7952446f0d2 100644
--- a/extensions/configuration-editing/schemas/attachContainer.schema.json
+++ b/extensions/configuration-editing/schemas/attachContainer.schema.json
@@ -1,7 +1,8 @@
{
- "$schema": "http://json-schema.org/schema#",
+ "$schema": "http://json-schema.org/draft-07/schema#",
"description": "Configures an attached to container",
"allowComments": true,
+ "allowTrailingCommas": true,
"type": "object",
"definitions": {
"attachContainer": {
@@ -18,9 +19,49 @@
"type": "integer"
}
},
+ "settings": {
+ "$ref": "vscode://schemas/settings/machine",
+ "description": "Machine specific settings that should be copied into the container."
+ },
+ "remoteEnv": {
+ "type": "object",
+ "additionalProperties": {
+ "type": [
+ "string",
+ "null"
+ ]
+ },
+ "description": "Remote environment variables."
+ },
+ "remoteUser": {
+ "type": "string",
+ "description": "The user VS Code Server will be started with. The default is the same user as the container."
+ },
"extensions": {
"type": "array",
"description": "An array of extensions that should be installed into the container.",
+ "items": {
+ "type": "string",
+ "pattern": "^([a-z0-9A-Z][a-z0-9\\-A-Z]*)\\.([a-z0-9A-Z][a-z0-9\\-A-Z]*)(@(0|[1-9]\\d*)\\.(0|[1-9]\\d*)\\.(0|[1-9]\\d*)(?:-((?:0|[1-9]\\d*|\\d*[a-zA-Z-][0-9a-zA-Z-]*)(?:\\.(?:0|[1-9]\\d*|\\d*[a-zA-Z-][0-9a-zA-Z-]*))*))?(?:\\+([0-9a-zA-Z-]+(?:\\.[0-9a-zA-Z-]+)*))?)?$",
+ "errorMessage": "Expected format: '${publisher}.${name}' or '${publisher}.${name}@${version}'. Example: 'ms-dotnettools.csharp'."
+ }
+ },
+ "userEnvProbe": {
+ "type": "string",
+ "enum": [
+ "none",
+ "loginShell",
+ "loginInteractiveShell",
+ "interactiveShell"
+ ],
+ "description": "User environment probe to run. The default is none."
+ },
+ "postAttachCommand": {
+ "type": [
+ "string",
+ "array"
+ ],
+ "description": "A command to run after attaching to the container. If this is a single string, it will be run in a shell. If this is an array of strings, it will be run as a single command without shell.",
"items": {
"type": "string"
}
diff --git a/extensions/configuration-editing/schemas/devContainer.schema.json b/extensions/configuration-editing/schemas/devContainer.schema.json
index 1358c74f2d2a9..b37e07fa65ceb 100644
--- a/extensions/configuration-editing/schemas/devContainer.schema.json
+++ b/extensions/configuration-editing/schemas/devContainer.schema.json
@@ -1,7 +1,8 @@
{
- "$schema": "http://json-schema.org/schema#",
+ "$schema": "http://json-schema.org/draft-07/schema#",
"description": "Defines a dev container",
"allowComments": true,
+ "allowTrailingCommas": true,
"type": "object",
"definitions": {
"devContainerCommon": {
@@ -15,13 +16,48 @@
"type": "array",
"description": "An array of extensions that should be installed into the container.",
"items": {
- "type": "string"
+ "type": "string",
+ "pattern": "^([a-z0-9A-Z][a-z0-9\\-A-Z]*)\\.([a-z0-9A-Z][a-z0-9\\-A-Z]*)(@(0|[1-9]\\d*)\\.(0|[1-9]\\d*)\\.(0|[1-9]\\d*)(?:-((?:0|[1-9]\\d*|\\d*[a-zA-Z-][0-9a-zA-Z-]*)(?:\\.(?:0|[1-9]\\d*|\\d*[a-zA-Z-][0-9a-zA-Z-]*))*))?(?:\\+([0-9a-zA-Z-]+(?:\\.[0-9a-zA-Z-]+)*))?)?$",
+ "errorMessage": "Expected format: '${publisher}.${name}' or '${publisher}.${name}@${version}'. Example: 'ms-dotnettools.csharp'."
}
},
"settings": {
"$ref": "vscode://schemas/settings/machine",
"description": "Machine specific settings that should be copied into the container."
},
+ "forwardPorts": {
+ "type": "array",
+ "description": "Ports that are forwarded from the container to the local machine.",
+ "items": {
+ "type": "integer",
+ "maximum": 65535,
+ "minimum": 0
+ }
+ },
+ "remoteEnv": {
+ "type": "object",
+ "additionalProperties": {
+ "type": [
+ "string",
+ "null"
+ ]
+ },
+ "description": "Remote environment variables."
+ },
+ "remoteUser": {
+ "type": "string",
+ "description": "The user VS Code Server will be started with. The default is the same user as the container."
+ },
+ "initializeCommand": {
+ "type": [
+ "string",
+ "array"
+ ],
+ "description": "A command to run locally before anything else. If this is a single string, it will be run in a shell. If this is an array of strings, it will be run as a single command without shell.",
+ "items": {
+ "type": "string"
+ }
+ },
"postCreateCommand": {
"type": [
"string",
@@ -32,9 +68,43 @@
"type": "string"
}
},
+ "postStartCommand": {
+ "type": [
+ "string",
+ "array"
+ ],
+ "description": "A command to run after starting the container. If this is a single string, it will be run in a shell. If this is an array of strings, it will be run as a single command without shell.",
+ "items": {
+ "type": "string"
+ }
+ },
+ "postAttachCommand": {
+ "type": [
+ "string",
+ "array"
+ ],
+ "description": "A command to run after attaching to the container. If this is a single string, it will be run in a shell. If this is an array of strings, it will be run as a single command without shell.",
+ "items": {
+ "type": "string"
+ }
+ },
"devPort": {
"type": "integer",
"description": "The port VS Code can use to connect to its backend."
+ },
+ "userEnvProbe": {
+ "type": "string",
+ "enum": [
+ "none",
+ "loginShell",
+ "loginInteractiveShell",
+ "interactiveShell"
+ ],
+ "description": "User environment probe to run. The default is none."
+ },
+ "codespaces": {
+ "type": "object",
+ "description": "Codespaces-specific configuration."
}
}
},
@@ -55,6 +125,28 @@
]
}
},
+ "containerEnv": {
+ "type": "object",
+ "additionalProperties": {
+ "type": "string"
+ },
+ "description": "Container environment variables."
+ },
+ "containerUser": {
+ "type": "string",
+ "description": "The user the container will be started with. The default is the user on the Docker image."
+ },
+ "updateRemoteUserUID": {
+ "type": "boolean",
+ "description": "Controls whether on Linux the container's user should be updated with the local user's UID and GID. On by default."
+ },
+ "mounts": {
+ "type": "array",
+ "description": "Mount points to set up when creating the container. See Docker's documentation for the --mount option for the supported syntax.",
+ "items": {
+ "type": "string"
+ }
+ },
"runArgs": {
"type": "array",
"description": "The arguments required when starting in the container.",
@@ -84,21 +176,89 @@
}
}
},
- "dockerFileContainer": {
+ "dockerfileContainer": {
+ "oneOf": [
+ {
+ "type": "object",
+ "properties": {
+ "build": {
+ "type": "object",
+ "description": "Docker build-related options.",
+ "allOf": [
+ {
+ "type": "object",
+ "properties": {
+ "dockerfile": {
+ "type": "string",
+ "description": "The location of the Dockerfile that defines the contents of the container. The path is relative to the folder containing the `devcontainer.json` file."
+ },
+ "context": {
+ "type": "string",
+ "description": "The location of the context folder for building the Docker image. The path is relative to the folder containing the `devcontainer.json` file."
+ }
+ },
+ "required": [
+ "dockerfile"
+ ]
+ },
+ {
+ "$ref": "#/definitions/buildOptions"
+ }
+ ]
+ }
+ },
+ "required": [
+ "build"
+ ]
+ },
+ {
+ "allOf": [
+ {
+ "type": "object",
+ "properties": {
+ "dockerFile": {
+ "type": "string",
+ "description": "The location of the Dockerfile that defines the contents of the container. The path is relative to the folder containing the `devcontainer.json` file."
+ },
+ "context": {
+ "type": "string",
+ "description": "The location of the context folder for building the Docker image. The path is relative to the folder containing the `devcontainer.json` file."
+ }
+ },
+ "required": [
+ "dockerFile"
+ ]
+ },
+ {
+ "type": "object",
+ "properties": {
+ "build": {
+ "description": "Docker build-related options.",
+ "$ref": "#/definitions/buildOptions"
+ }
+ }
+ }
+ ]
+ }
+ ]
+ },
+ "buildOptions": {
"type": "object",
"properties": {
- "dockerFile": {
+ "target": {
"type": "string",
- "description": "The location of the Dockerfile that defines the contents of the container. The path is relative to the folder containing the `devcontainer.json` file."
+ "description": "Target stage in a multi-stage build."
},
- "context": {
- "type": "string",
- "description": "The location of the context folder for building the Docker image. The path is relative to the folder containing the `devcontainer.json` file."
+ "args": {
+ "type": "object",
+ "additionalProperties": {
+ "type": [
+ "string"
+ ]
+ },
+ "description": "Build arguments."
}
- },
- "required": [
- "dockerFile"
- ]
+ }
},
"imageContainer": {
"type": "object",
@@ -156,33 +316,42 @@
]
}
},
- "allOf": [
+ "oneOf": [
{
- "oneOf": [
+ "allOf": [
{
- "allOf": [
+ "oneOf": [
{
- "oneOf": [
+ "allOf": [
{
- "$ref": "#/definitions/dockerFileContainer"
+ "oneOf": [
+ {
+ "$ref": "#/definitions/dockerfileContainer"
+ },
+ {
+ "$ref": "#/definitions/imageContainer"
+ }
+ ]
},
{
- "$ref": "#/definitions/imageContainer"
+ "$ref": "#/definitions/nonComposeBase"
}
]
},
{
- "$ref": "#/definitions/nonComposeBase"
+ "$ref": "#/definitions/composeContainer"
}
]
},
{
- "$ref": "#/definitions/composeContainer"
+ "$ref": "#/definitions/devContainerCommon"
}
]
},
{
- "$ref": "#/definitions/devContainerCommon"
+ "type": "object",
+ "$ref": "#/definitions/devContainerCommon",
+ "additionalProperties": false
}
]
}
diff --git a/extensions/configuration-editing/src/configurationEditingMain.ts b/extensions/configuration-editing/src/configurationEditingMain.ts
new file mode 100644
index 0000000000000..966073e23f87b
--- /dev/null
+++ b/extensions/configuration-editing/src/configurationEditingMain.ts
@@ -0,0 +1,138 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import { getLocation, parse, visit } from 'jsonc-parser';
+import * as vscode from 'vscode';
+import * as nls from 'vscode-nls';
+import { SettingsDocument } from './settingsDocumentHelper';
+import { provideInstalledExtensionProposals } from './extensionsProposals';
+const localize = nls.loadMessageBundle();
+
+export function activate(context: vscode.ExtensionContext): void {
+ //settings.json suggestions
+ context.subscriptions.push(registerSettingsCompletions());
+
+ //extensions suggestions
+ context.subscriptions.push(...registerExtensionsCompletions());
+
+ // launch.json variable suggestions
+ context.subscriptions.push(registerVariableCompletions('**/launch.json'));
+
+ // task.json variable suggestions
+ context.subscriptions.push(registerVariableCompletions('**/tasks.json'));
+}
+
+function registerSettingsCompletions(): vscode.Disposable {
+ return vscode.languages.registerCompletionItemProvider({ language: 'jsonc', pattern: '**/settings.json' }, {
+ provideCompletionItems(document, position, token) {
+ return new SettingsDocument(document).provideCompletionItems(position, token);
+ }
+ });
+}
+
+function registerVariableCompletions(pattern: string): vscode.Disposable {
+ return vscode.languages.registerCompletionItemProvider({ language: 'jsonc', pattern }, {
+ provideCompletionItems(document, position, _token) {
+ const location = getLocation(document.getText(), document.offsetAt(position));
+ if (!location.isAtPropertyKey && location.previousNode && location.previousNode.type === 'string') {
+ const indexOf$ = document.lineAt(position.line).text.indexOf('$');
+ const startPosition = indexOf$ >= 0 ? new vscode.Position(position.line, indexOf$) : position;
+
+ return [
+ { label: 'workspaceFolder', detail: localize('workspaceFolder', "The path of the folder opened in VS Code") },
+ { label: 'workspaceFolderBasename', detail: localize('workspaceFolderBasename', "The name of the folder opened in VS Code without any slashes (/)") },
+ { label: 'relativeFile', detail: localize('relativeFile', "The current opened file relative to ${workspaceFolder}") },
+ { label: 'relativeFileDirname', detail: localize('relativeFileDirname', "The current opened file's dirname relative to ${workspaceFolder}") },
+ { label: 'file', detail: localize('file', "The current opened file") },
+ { label: 'cwd', detail: localize('cwd', "The task runner's current working directory on startup") },
+ { label: 'lineNumber', detail: localize('lineNumber', "The current selected line number in the active file") },
+ { label: 'selectedText', detail: localize('selectedText', "The current selected text in the active file") },
+ { label: 'fileDirname', detail: localize('fileDirname', "The current opened file's dirname") },
+ { label: 'fileExtname', detail: localize('fileExtname', "The current opened file's extension") },
+ { label: 'fileBasename', detail: localize('fileBasename', "The current opened file's basename") },
+ { label: 'fileBasenameNoExtension', detail: localize('fileBasenameNoExtension', "The current opened file's basename with no file extension") },
+ { label: 'defaultBuildTask', detail: localize('defaultBuildTask', "The name of the default build task. If there is not a single default build task then a quick pick is shown to choose the build task.") },
+ ].map(variable => ({
+ label: '${' + variable.label + '}',
+ range: new vscode.Range(startPosition, position),
+ detail: variable.detail
+ }));
+ }
+
+ return [];
+ }
+ });
+}
+
+interface IExtensionsContent {
+ recommendations: string[];
+}
+
+function registerExtensionsCompletions(): vscode.Disposable[] {
+ return [registerExtensionsCompletionsInExtensionsDocument(), registerExtensionsCompletionsInWorkspaceConfigurationDocument()];
+}
+
+function registerExtensionsCompletionsInExtensionsDocument(): vscode.Disposable {
+ return vscode.languages.registerCompletionItemProvider({ pattern: '**/extensions.json' }, {
+ provideCompletionItems(document, position, _token) {
+ const location = getLocation(document.getText(), document.offsetAt(position));
+ const range = document.getWordRangeAtPosition(position) || new vscode.Range(position, position);
+ if (location.path[0] === 'recommendations') {
+ const extensionsContent = parse(document.getText());
+ return provideInstalledExtensionProposals(extensionsContent && extensionsContent.recommendations || [], range, false);
+ }
+ return [];
+ }
+ });
+}
+
+function registerExtensionsCompletionsInWorkspaceConfigurationDocument(): vscode.Disposable {
+ return vscode.languages.registerCompletionItemProvider({ pattern: '**/*.code-workspace' }, {
+ provideCompletionItems(document, position, _token) {
+ const location = getLocation(document.getText(), document.offsetAt(position));
+ const range = document.getWordRangeAtPosition(position) || new vscode.Range(position, position);
+ if (location.path[0] === 'extensions' && location.path[1] === 'recommendations') {
+ const extensionsContent = parse(document.getText())['extensions'];
+ return provideInstalledExtensionProposals(extensionsContent && extensionsContent.recommendations || [], range, false);
+ }
+ return [];
+ }
+ });
+}
+
+vscode.languages.registerDocumentSymbolProvider({ pattern: '**/launch.json', language: 'jsonc' }, {
+ provideDocumentSymbols(document: vscode.TextDocument, _token: vscode.CancellationToken): vscode.ProviderResult {
+ const result: vscode.SymbolInformation[] = [];
+ let name: string = '';
+ let lastProperty = '';
+ let startOffset = 0;
+ let depthInObjects = 0;
+
+ visit(document.getText(), {
+ onObjectProperty: (property, _offset, _length) => {
+ lastProperty = property;
+ },
+ onLiteralValue: (value: any, _offset: number, _length: number) => {
+ if (lastProperty === 'name') {
+ name = value;
+ }
+ },
+ onObjectBegin: (offset: number, _length: number) => {
+ depthInObjects++;
+ if (depthInObjects === 2) {
+ startOffset = offset;
+ }
+ },
+ onObjectEnd: (offset: number, _length: number) => {
+ if (name && depthInObjects === 2) {
+ result.push(new vscode.SymbolInformation(name, vscode.SymbolKind.Object, new vscode.Range(document.positionAt(startOffset), document.positionAt(offset))));
+ }
+ depthInObjects--;
+ },
+ });
+
+ return result;
+ }
+}, { label: 'Launch Targets' });
diff --git a/extensions/configuration-editing/src/extension.ts b/extensions/configuration-editing/src/extension.ts
deleted file mode 100644
index 72e2fcf627f94..0000000000000
--- a/extensions/configuration-editing/src/extension.ts
+++ /dev/null
@@ -1,222 +0,0 @@
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-
-import { getLocation, parse, visit } from 'jsonc-parser';
-import * as path from 'path';
-import * as vscode from 'vscode';
-import * as nls from 'vscode-nls';
-import { SettingsDocument } from './settingsDocumentHelper';
-const localize = nls.loadMessageBundle();
-
-const fadedDecoration = vscode.window.createTextEditorDecorationType({
- light: {
- color: '#757575'
- },
- dark: {
- color: '#878787'
- }
-});
-
-let pendingLaunchJsonDecoration: NodeJS.Timer;
-
-export function activate(context: vscode.ExtensionContext): void {
- //settings.json suggestions
- context.subscriptions.push(registerSettingsCompletions());
-
- //extensions suggestions
- context.subscriptions.push(...registerExtensionsCompletions());
-
- // launch.json variable suggestions
- context.subscriptions.push(registerVariableCompletions('**/launch.json'));
-
- // task.json variable suggestions
- context.subscriptions.push(registerVariableCompletions('**/tasks.json'));
-
- // launch.json decorations
- context.subscriptions.push(vscode.window.onDidChangeActiveTextEditor(editor => updateLaunchJsonDecorations(editor), null, context.subscriptions));
- context.subscriptions.push(vscode.workspace.onDidChangeTextDocument(event => {
- if (vscode.window.activeTextEditor && event.document === vscode.window.activeTextEditor.document) {
- if (pendingLaunchJsonDecoration) {
- clearTimeout(pendingLaunchJsonDecoration);
- }
- pendingLaunchJsonDecoration = setTimeout(() => updateLaunchJsonDecorations(vscode.window.activeTextEditor), 1000);
- }
- }, null, context.subscriptions));
- updateLaunchJsonDecorations(vscode.window.activeTextEditor);
-}
-
-function registerSettingsCompletions(): vscode.Disposable {
- return vscode.languages.registerCompletionItemProvider({ language: 'jsonc', pattern: '**/settings.json' }, {
- provideCompletionItems(document, position, token) {
- return new SettingsDocument(document).provideCompletionItems(position, token);
- }
- });
-}
-
-function registerVariableCompletions(pattern: string): vscode.Disposable {
- return vscode.languages.registerCompletionItemProvider({ language: 'jsonc', pattern }, {
- provideCompletionItems(document, position, _token) {
- const location = getLocation(document.getText(), document.offsetAt(position));
- if (!location.isAtPropertyKey && location.previousNode && location.previousNode.type === 'string') {
- const indexOf$ = document.lineAt(position.line).text.indexOf('$');
- const startPosition = indexOf$ >= 0 ? new vscode.Position(position.line, indexOf$) : position;
-
- return [
- { label: 'workspaceFolder', detail: localize('workspaceFolder', "The path of the folder opened in VS Code") },
- { label: 'workspaceFolderBasename', detail: localize('workspaceFolderBasename', "The name of the folder opened in VS Code without any slashes (/)") },
- { label: 'relativeFile', detail: localize('relativeFile', "The current opened file relative to ${workspaceFolder}") },
- { label: 'relativeFileDirname', detail: localize('relativeFileDirname', "The current opened file's dirname relative to ${workspaceFolder}") },
- { label: 'file', detail: localize('file', "The current opened file") },
- { label: 'cwd', detail: localize('cwd', "The task runner's current working directory on startup") },
- { label: 'lineNumber', detail: localize('lineNumber', "The current selected line number in the active file") },
- { label: 'selectedText', detail: localize('selectedText', "The current selected text in the active file") },
- { label: 'fileDirname', detail: localize('fileDirname', "The current opened file's dirname") },
- { label: 'fileExtname', detail: localize('fileExtname', "The current opened file's extension") },
- { label: 'fileBasename', detail: localize('fileBasename', "The current opened file's basename") },
- { label: 'fileBasenameNoExtension', detail: localize('fileBasenameNoExtension', "The current opened file's basename with no file extension") },
- { label: 'defaultBuildTask', detail: localize('defaultBuildTask', "The name of the default build task. If there is not a single default build task then a quick pick is shown to choose the build task.") },
- ].map(variable => ({
- label: '${' + variable.label + '}',
- range: new vscode.Range(startPosition, position),
- detail: variable.detail
- }));
- }
-
- return [];
- }
- });
-}
-
-interface IExtensionsContent {
- recommendations: string[];
-}
-
-function registerExtensionsCompletions(): vscode.Disposable[] {
- return [registerExtensionsCompletionsInExtensionsDocument(), registerExtensionsCompletionsInWorkspaceConfigurationDocument()];
-}
-
-function registerExtensionsCompletionsInExtensionsDocument(): vscode.Disposable {
- return vscode.languages.registerCompletionItemProvider({ pattern: '**/extensions.json' }, {
- provideCompletionItems(document, position, _token) {
- const location = getLocation(document.getText(), document.offsetAt(position));
- const range = document.getWordRangeAtPosition(position) || new vscode.Range(position, position);
- if (location.path[0] === 'recommendations') {
- const extensionsContent = parse(document.getText());
- return provideInstalledExtensionProposals(extensionsContent, range);
- }
- return [];
- }
- });
-}
-
-function registerExtensionsCompletionsInWorkspaceConfigurationDocument(): vscode.Disposable {
- return vscode.languages.registerCompletionItemProvider({ pattern: '**/*.code-workspace' }, {
- provideCompletionItems(document, position, _token) {
- const location = getLocation(document.getText(), document.offsetAt(position));
- const range = document.getWordRangeAtPosition(position) || new vscode.Range(position, position);
- if (location.path[0] === 'extensions' && location.path[1] === 'recommendations') {
- const extensionsContent = parse(document.getText())['extensions'];
- return provideInstalledExtensionProposals(extensionsContent, range);
- }
- return [];
- }
- });
-}
-
-function provideInstalledExtensionProposals(extensionsContent: IExtensionsContent, range: vscode.Range): vscode.ProviderResult {
- const alreadyEnteredExtensions = extensionsContent && extensionsContent.recommendations || [];
- if (Array.isArray(alreadyEnteredExtensions)) {
- const knownExtensionProposals = vscode.extensions.all.filter(e =>
- !(e.id.startsWith('vscode.')
- || e.id === 'Microsoft.vscode-markdown'
- || alreadyEnteredExtensions.indexOf(e.id) > -1));
- if (knownExtensionProposals.length) {
- return knownExtensionProposals.map(e => {
- const item = new vscode.CompletionItem(e.id);
- const insertText = `"${e.id}"`;
- item.kind = vscode.CompletionItemKind.Value;
- item.insertText = insertText;
- item.range = range;
- item.filterText = insertText;
- return item;
- });
- } else {
- const example = new vscode.CompletionItem(localize('exampleExtension', "Example"));
- example.insertText = '"vscode.csharp"';
- example.kind = vscode.CompletionItemKind.Value;
- example.range = range;
- return [example];
- }
- }
- return undefined;
-}
-
-function updateLaunchJsonDecorations(editor: vscode.TextEditor | undefined): void {
- if (!editor || path.basename(editor.document.fileName) !== 'launch.json') {
- return;
- }
-
- const ranges: vscode.Range[] = [];
- let addPropertyAndValue = false;
- let depthInArray = 0;
- visit(editor.document.getText(), {
- onObjectProperty: (property, offset, length) => {
- // Decorate attributes which are unlikely to be edited by the user.
- // Only decorate "configurations" if it is not inside an array (compounds have a configurations property which should not be decorated).
- addPropertyAndValue = property === 'version' || property === 'type' || property === 'request' || property === 'compounds' || (property === 'configurations' && depthInArray === 0);
- if (addPropertyAndValue) {
- ranges.push(new vscode.Range(editor.document.positionAt(offset), editor.document.positionAt(offset + length)));
- }
- },
- onLiteralValue: (_value, offset, length) => {
- if (addPropertyAndValue) {
- ranges.push(new vscode.Range(editor.document.positionAt(offset), editor.document.positionAt(offset + length)));
- }
- },
- onArrayBegin: (_offset: number, _length: number) => {
- depthInArray++;
- },
- onArrayEnd: (_offset: number, _length: number) => {
- depthInArray--;
- }
- });
-
- editor.setDecorations(fadedDecoration, ranges);
-}
-
-vscode.languages.registerDocumentSymbolProvider({ pattern: '**/launch.json', language: 'jsonc' }, {
- provideDocumentSymbols(document: vscode.TextDocument, _token: vscode.CancellationToken): vscode.ProviderResult {
- const result: vscode.SymbolInformation[] = [];
- let name: string = '';
- let lastProperty = '';
- let startOffset = 0;
- let depthInObjects = 0;
-
- visit(document.getText(), {
- onObjectProperty: (property, _offset, _length) => {
- lastProperty = property;
- },
- onLiteralValue: (value: any, _offset: number, _length: number) => {
- if (lastProperty === 'name') {
- name = value;
- }
- },
- onObjectBegin: (offset: number, _length: number) => {
- depthInObjects++;
- if (depthInObjects === 2) {
- startOffset = offset;
- }
- },
- onObjectEnd: (offset: number, _length: number) => {
- if (name && depthInObjects === 2) {
- result.push(new vscode.SymbolInformation(name, vscode.SymbolKind.Object, new vscode.Range(document.positionAt(startOffset), document.positionAt(offset))));
- }
- depthInObjects--;
- },
- });
-
- return result;
- }
-}, { label: 'Launch Targets' });
diff --git a/extensions/configuration-editing/src/extensionsProposals.ts b/extensions/configuration-editing/src/extensionsProposals.ts
new file mode 100644
index 0000000000000..60533ae29759e
--- /dev/null
+++ b/extensions/configuration-editing/src/extensionsProposals.ts
@@ -0,0 +1,35 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import * as vscode from 'vscode';
+import * as nls from 'vscode-nls';
+const localize = nls.loadMessageBundle();
+
+
+export function provideInstalledExtensionProposals(existing: string[], range: vscode.Range, includeBuiltinExtensions: boolean): vscode.ProviderResult {
+ if (Array.isArray(existing)) {
+ const extensions = includeBuiltinExtensions ? vscode.extensions.all : vscode.extensions.all.filter(e => !(e.id.startsWith('vscode.') || e.id === 'Microsoft.vscode-markdown'));
+ const knownExtensionProposals = extensions.filter(e => existing.indexOf(e.id) === -1);
+ if (knownExtensionProposals.length) {
+ return knownExtensionProposals.map(e => {
+ const item = new vscode.CompletionItem(e.id);
+ const insertText = `"${e.id}"`;
+ item.kind = vscode.CompletionItemKind.Value;
+ item.insertText = insertText;
+ item.range = range;
+ item.filterText = insertText;
+ return item;
+ });
+ } else {
+ const example = new vscode.CompletionItem(localize('exampleExtension', "Example"));
+ example.insertText = '"vscode.csharp"';
+ example.kind = vscode.CompletionItemKind.Value;
+ example.range = range;
+ return [example];
+ }
+ }
+ return undefined;
+}
+
diff --git a/extensions/configuration-editing/src/settingsDocumentHelper.ts b/extensions/configuration-editing/src/settingsDocumentHelper.ts
index 970f2a5ca18c3..5e466c2eb6fa7 100644
--- a/extensions/configuration-editing/src/settingsDocumentHelper.ts
+++ b/extensions/configuration-editing/src/settingsDocumentHelper.ts
@@ -4,8 +4,9 @@
*--------------------------------------------------------------------------------------------*/
import * as vscode from 'vscode';
-import { getLocation, Location } from 'jsonc-parser';
+import { getLocation, Location, parse } from 'jsonc-parser';
import * as nls from 'vscode-nls';
+import { provideInstalledExtensionProposals } from './extensionsProposals';
const localize = nls.loadMessageBundle();
@@ -13,7 +14,7 @@ export class SettingsDocument {
constructor(private document: vscode.TextDocument) { }
- public provideCompletionItems(position: vscode.Position, _token: vscode.CancellationToken): vscode.ProviderResult {
+ public provideCompletionItems(position: vscode.Position, _token: vscode.CancellationToken): vscode.ProviderResult {
const location = getLocation(this.document.getText(), this.document.offsetAt(position));
const range = this.document.getWordRangeAtPosition(position) || new vscode.Range(position, position);
@@ -34,7 +35,20 @@ export class SettingsDocument {
// files.defaultLanguage
if (location.path[0] === 'files.defaultLanguage') {
- return this.provideLanguageCompletionItems(location, range);
+ return this.provideLanguageCompletionItems(location, range).then(items => {
+
+ // Add special item '${activeEditorLanguage}'
+ return [this.newSimpleCompletionItem(JSON.stringify('${activeEditorLanguage}'), range, localize('activeEditor', "Use the language of the currently active text editor if any")), ...items];
+ });
+ }
+
+ // settingsSync.ignoredExtensions
+ if (location.path[0] === 'settingsSync.ignoredExtensions') {
+ let ignoredExtensions = [];
+ try {
+ ignoredExtensions = parse(this.document.getText())['settingsSync.ignoredExtensions'];
+ } catch (e) {/* ignore error */ }
+ return provideInstalledExtensionProposals(ignoredExtensions, range, true);
}
return this.provideLanguageOverridesCompletionItems(location, position);
@@ -82,7 +96,7 @@ export class SettingsDocument {
}));
} else {
// Value
- return this.provideLanguageCompletionItems(location, range);
+ return this.provideLanguageCompletionItemsForLanguageOverrides(location, range);
}
}
@@ -153,7 +167,12 @@ export class SettingsDocument {
return Promise.resolve(completions);
}
- private provideLanguageCompletionItems(_location: Location, range: vscode.Range, formatFunc: (string: string) => string = (l) => JSON.stringify(l)): vscode.ProviderResult {
+ private provideLanguageCompletionItems(_location: Location, range: vscode.Range, formatFunc: (string: string) => string = (l) => JSON.stringify(l)): Thenable {
+ return vscode.languages.getLanguages()
+ .then(languages => languages.map(l => this.newSimpleCompletionItem(formatFunc(l), range)));
+ }
+
+ private provideLanguageCompletionItemsForLanguageOverrides(_location: Location, range: vscode.Range, formatFunc: (string: string) => string = (l) => JSON.stringify(l)): Thenable {
return vscode.languages.getLanguages().then(languages => {
const completionItems = [];
const configuration = vscode.workspace.getConfiguration();
@@ -178,7 +197,7 @@ export class SettingsDocument {
let text = this.document.getText(range);
if (text && text.trim().startsWith('[')) {
range = new vscode.Range(new vscode.Position(range.start.line, range.start.character + text.indexOf('[')), range.end);
- return this.provideLanguageCompletionItems(location, range, language => `"[${language}]"`);
+ return this.provideLanguageCompletionItemsForLanguageOverrides(location, range, language => `"[${language}]"`);
}
range = this.document.getWordRangeAtPosition(position) || new vscode.Range(position, position);
@@ -204,8 +223,8 @@ export class SettingsDocument {
if (location.path.length === 1 && location.previousNode && typeof location.previousNode.value === 'string' && location.previousNode.value.startsWith('[')) {
// Suggestion model word matching includes closed sqaure bracket and ending quote
// Hence include them in the proposal to replace
- let range = this.document.getWordRangeAtPosition(position) || new vscode.Range(position, position);
- return this.provideLanguageCompletionItems(location, range, language => `"[${language}]"`);
+ const range = this.document.getWordRangeAtPosition(position) || new vscode.Range(position, position);
+ return this.provideLanguageCompletionItemsForLanguageOverrides(location, range, language => `"[${language}]"`);
}
return Promise.resolve([]);
}
diff --git a/extensions/configuration-editing/yarn.lock b/extensions/configuration-editing/yarn.lock
index d5aafed1189df..36aab5fd224dc 100644
--- a/extensions/configuration-editing/yarn.lock
+++ b/extensions/configuration-editing/yarn.lock
@@ -7,12 +7,12 @@
resolved "https://registry.yarnpkg.com/@types/node/-/node-12.11.7.tgz#57682a9771a3f7b09c2497f28129a0462966524a"
integrity sha512-JNbGaHFCLwgHn/iCckiGSOZ1XYHsKFwREtzPwSGCVld1SGhOlmZw2D4ZI94HQCrBHbADzW9m4LER/8olJTRGHA==
-jsonc-parser@^2.1.1:
- version "2.1.1"
- resolved "https://registry.yarnpkg.com/jsonc-parser/-/jsonc-parser-2.1.1.tgz#83dc3d7a6e7186346b889b1280eefa04446c6d3e"
- integrity sha512-VC0CjnWJylKB1iov4u76/W/5Ef0ydDkjtYWxoZ9t3HdWlSnZQwZL5MgFikaB/EtQ4RmMEw3tmQzuYnZA2/Ja1g==
+jsonc-parser@^2.2.1:
+ version "2.2.1"
+ resolved "https://registry.yarnpkg.com/jsonc-parser/-/jsonc-parser-2.2.1.tgz#db73cd59d78cce28723199466b2a03d1be1df2bc"
+ integrity sha512-o6/yDBYccGvTz1+QFevz6l6OBZ2+fMVu2JZ9CIhzsYRX4mjaK5IyX9eldUdCmga16zlgQxyrj5pt9kzuj2C02w==
-vscode-nls@^4.0.0:
- version "4.0.0"
- resolved "https://registry.yarnpkg.com/vscode-nls/-/vscode-nls-4.0.0.tgz#4001c8a6caba5cedb23a9c5ce1090395c0e44002"
- integrity sha512-qCfdzcH+0LgQnBpZA53bA32kzp9rpq/f66Som577ObeuDlFIrtbEJ+A/+CCxjIh4G8dpJYNCKIsxpRAHIfsbNw==
+vscode-nls@^4.1.1:
+ version "4.1.1"
+ resolved "https://registry.yarnpkg.com/vscode-nls/-/vscode-nls-4.1.1.tgz#f9916b64e4947b20322defb1e676a495861f133c"
+ integrity sha512-4R+2UoUUU/LdnMnFjePxfLqNhBS8lrAFyX7pjb2ud/lqDkrUavFUTcG7wR0HBZFakae0Q6KLBFjMS6W93F403A==
diff --git a/extensions/cpp/cgmanifest.json b/extensions/cpp/cgmanifest.json
index 3dc984e58c2f0..070312a63e4fa 100644
--- a/extensions/cpp/cgmanifest.json
+++ b/extensions/cpp/cgmanifest.json
@@ -6,11 +6,11 @@
"git": {
"name": "jeff-hykin/cpp-textmate-grammar",
"repositoryUrl": "https://github.com/jeff-hykin/cpp-textmate-grammar",
- "commitHash": "d937b80a19706d518e5fe52357d7c50d34a26e60"
+ "commitHash": "666808cab3907fc91ed4d3901060ee6b045cca58"
}
},
"license": "MIT",
- "version": "1.14.11",
+ "version": "1.14.15",
"description": "The files syntaxes/c.json and syntaxes/c++.json were derived from https://github.com/atom/language-c which was originally converted from the C TextMate bundle https://github.com/textmate/c.tmbundle."
},
{
@@ -19,7 +19,7 @@
"git": {
"name": "textmate/c.tmbundle",
"repositoryUrl": "https://github.com/textmate/c.tmbundle",
- "commitHash": "9aa365882274ca52f01722f3dbb169b9539a20ee"
+ "commitHash": "60daf83b9d45329524f7847a75e9298b3aae5805"
}
},
"licenseDetail": [
diff --git a/extensions/cpp/package.json b/extensions/cpp/package.json
index c3ed8538bd8c8..1c8a244d28596 100644
--- a/extensions/cpp/package.json
+++ b/extensions/cpp/package.json
@@ -34,7 +34,8 @@
".c++",
".hpp",
".hh",
- ".hxx",
+ ".hxx",
+ ".h++",
".h",
".ii",
".ino",
@@ -75,11 +76,11 @@
"snippets": [
{
"language": "c",
- "path": "./snippets/c.json"
+ "path": "./snippets/c.code-snippets"
},
{
"language": "cpp",
- "path": "./snippets/cpp.json"
+ "path": "./snippets/cpp.code-snippets"
}
]
}
diff --git a/extensions/cpp/snippets/c.json b/extensions/cpp/snippets/c.code-snippets
similarity index 100%
rename from extensions/cpp/snippets/c.json
rename to extensions/cpp/snippets/c.code-snippets
diff --git a/extensions/cpp/snippets/cpp.json b/extensions/cpp/snippets/cpp.code-snippets
similarity index 100%
rename from extensions/cpp/snippets/cpp.json
rename to extensions/cpp/snippets/cpp.code-snippets
diff --git a/extensions/cpp/syntaxes/c.tmLanguage.json b/extensions/cpp/syntaxes/c.tmLanguage.json
index d8c4dca256bab..eef07eeb53d85 100644
--- a/extensions/cpp/syntaxes/c.tmLanguage.json
+++ b/extensions/cpp/syntaxes/c.tmLanguage.json
@@ -4,7 +4,7 @@
"If you want to provide a fix or improvement, please create a pull request against the original repository.",
"Once accepted there, we are happy to receive an update request."
],
- "version": "https://github.com/jeff-hykin/cpp-textmate-grammar/commit/d937b80a19706d518e5fe52357d7c50d34a26e60",
+ "version": "https://github.com/jeff-hykin/cpp-textmate-grammar/commit/72b309aabb63bf14a3cdf0280149121db005d616",
"name": "C",
"scopeName": "source.c",
"patterns": [
@@ -583,7 +583,7 @@
},
"case_statement": {
"name": "meta.conditional.case.c",
- "begin": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\*|->)))((?:[a-zA-Z_]\\w*\\s*(?:(?:(?:\\.\\*|\\.))|(?:(?:->\\*|->)))\\s*)*)\\s*(\\b(?!(?:atomic_uint_least64_t|atomic_uint_least16_t|atomic_uint_least32_t|atomic_uint_least8_t|atomic_int_least16_t|atomic_uint_fast64_t|atomic_uint_fast32_t|atomic_int_least64_t|atomic_int_least32_t|pthread_rwlockattr_t|atomic_uint_fast16_t|pthread_mutexattr_t|atomic_int_fast16_t|atomic_uint_fast8_t|atomic_int_fast64_t|atomic_int_least8_t|atomic_int_fast32_t|atomic_int_fast8_t|pthread_condattr_t|atomic_uintptr_t|atomic_ptrdiff_t|pthread_rwlock_t|atomic_uintmax_t|pthread_mutex_t|atomic_intmax_t|atomic_intptr_t|atomic_char32_t|atomic_char16_t|pthread_attr_t|atomic_wchar_t|uint_least64_t|uint_least32_t|uint_least16_t|pthread_cond_t|pthread_once_t|uint_fast64_t|uint_fast16_t|atomic_size_t|uint_least8_t|int_least64_t|int_least32_t|int_least16_t|pthread_key_t|atomic_ullong|atomic_ushort|uint_fast32_t|atomic_schar|atomic_short|uint_fast8_t|int_fast64_t|int_fast32_t|int_fast16_t|atomic_ulong|atomic_llong|int_least8_t|atomic_uchar|memory_order|suseconds_t|int_fast8_t|atomic_bool|atomic_char|atomic_uint|atomic_long|atomic_int|useconds_t|_Imaginary|uintptr_t|pthread_t|in_addr_t|blksize_t|in_port_t|uintmax_t|uintmax_t|blkcnt_t|uint16_t|unsigned|_Complex|uint32_t|intptr_t|intmax_t|intmax_t|uint64_t|u_quad_t|int64_t|int32_t|ssize_t|caddr_t|clock_t|uint8_t|u_short|swblk_t|segsz_t|int16_t|fixpt_t|daddr_t|nlink_t|qaddr_t|size_t|time_t|mode_t|signed|quad_t|ushort|u_long|u_char|double|int8_t|ino_t|uid_t|pid_t|_Bool|float|dev_t|div_t|short|gid_t|off_t|u_int|key_t|id_t|uint|long|void|char|bool|id_t|int)\\b)[a-zA-Z_]\\w*\\b(?!\\())",
+ "match": "((?:[a-zA-Z_]\\w*|(?<=\\]|\\)))\\s*)(?:((?:\\.\\*|\\.))|((?:->\\*|->)))((?:[a-zA-Z_]\\w*\\s*(?:(?:(?:\\.\\*|\\.))|(?:(?:->\\*|->)))\\s*)*)\\s*(\\b(?!(?:atomic_uint_least64_t|atomic_uint_least16_t|atomic_uint_least32_t|atomic_uint_least8_t|atomic_int_least16_t|atomic_uint_fast64_t|atomic_uint_fast32_t|atomic_int_least64_t|atomic_int_least32_t|pthread_rwlockattr_t|atomic_uint_fast16_t|pthread_mutexattr_t|atomic_int_fast16_t|atomic_uint_fast8_t|atomic_int_fast64_t|atomic_int_least8_t|atomic_int_fast32_t|atomic_int_fast8_t|pthread_condattr_t|atomic_uintptr_t|atomic_ptrdiff_t|pthread_rwlock_t|atomic_uintmax_t|pthread_mutex_t|atomic_intmax_t|atomic_intptr_t|atomic_char32_t|atomic_char16_t|pthread_attr_t|atomic_wchar_t|uint_least64_t|uint_least32_t|uint_least16_t|pthread_cond_t|pthread_once_t|uint_fast64_t|uint_fast16_t|atomic_size_t|uint_least8_t|int_least64_t|int_least32_t|int_least16_t|pthread_key_t|atomic_ullong|atomic_ushort|uint_fast32_t|atomic_schar|atomic_short|uint_fast8_t|int_fast64_t|int_fast32_t|int_fast16_t|atomic_ulong|atomic_llong|int_least8_t|atomic_uchar|memory_order|suseconds_t|int_fast8_t|atomic_bool|atomic_char|atomic_uint|atomic_long|atomic_int|useconds_t|_Imaginary|blksize_t|pthread_t|in_addr_t|uintptr_t|in_port_t|uintmax_t|uintmax_t|blkcnt_t|uint16_t|unsigned|_Complex|uint32_t|intptr_t|intmax_t|intmax_t|uint64_t|u_quad_t|int64_t|int32_t|ssize_t|caddr_t|clock_t|uint8_t|u_short|swblk_t|segsz_t|int16_t|fixpt_t|daddr_t|nlink_t|qaddr_t|size_t|time_t|mode_t|signed|quad_t|ushort|u_long|u_char|double|int8_t|ino_t|uid_t|pid_t|_Bool|float|dev_t|div_t|short|gid_t|off_t|u_int|key_t|id_t|uint|long|void|char|bool|id_t|int)\\b)[a-zA-Z_]\\w*\\b(?!\\())",
"captures": {
"1": {
"name": "variable.other.object.access.c"
@@ -2772,7 +2772,7 @@
}
},
"static_assert": {
- "begin": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"patterns": [
@@ -3051,7 +3051,7 @@
},
"switch_conditional_parentheses": {
"name": "meta.conditional.switch.c",
- "begin": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"patterns": [
@@ -3099,7 +3099,7 @@
},
"switch_statement": {
"name": "meta.block.switch.c",
- "begin": "(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(((?:protected|private|public))\\s*(:))",
+ "match": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(((?:protected|private|public))\\s*(:))",
"captures": {
"1": {
"patterns": [
@@ -194,7 +194,7 @@
},
"alignas_operator": {
"contentName": "meta.arguments.operator.alignas.cpp",
- "begin": "((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"name": "keyword.operator.functionlike.cpp keyword.operator.alignas.cpp"
@@ -242,7 +242,7 @@
},
"alignof_operator": {
"contentName": "meta.arguments.operator.alignof.cpp",
- "begin": "((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"name": "keyword.operator.functionlike.cpp keyword.operator.alignof.cpp"
@@ -442,7 +442,7 @@
}
},
"builtin_storage_type_initilizer": {
- "begin": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"patterns": [
@@ -624,7 +624,7 @@
},
"case_statement": {
"name": "meta.conditional.case.cpp",
- "begin": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(DLLEXPORT)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(final)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(:)((?>[^{]*)))?))",
+ "begin": "((((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(DLLEXPORT)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(final)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(:)((?>[^{]*)))?))",
"beginCaptures": {
"1": {
"name": "meta.head.class.cpp"
@@ -966,7 +966,7 @@
]
},
"class_declare": {
- "match": "(class)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))\\b(?!override\\W|override\\$|final\\W|final\\$)((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=\\S)(?![:{])",
+ "match": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))\\b(?!override\\W|override\\$|final\\W|final\\$)((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=\\S)(?![:{])",
"captures": {
"1": {
"name": "storage.type.class.declare.cpp"
@@ -1006,7 +1006,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -1425,7 +1425,7 @@
},
"constructor_inline": {
"name": "meta.function.definition.special.constructor.cpp",
- "begin": "(^((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?:constexpr|explicit|mutable|virtual|inline|friend)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:(?:constexpr|explicit|mutable|virtual|inline|friend)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(default)|(delete))",
+ "match": "(\\=)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(default)|(delete))",
"captures": {
"1": {
"name": "keyword.operator.assignment.cpp"
@@ -1733,7 +1733,7 @@
},
"constructor_root": {
"name": "meta.function.definition.special.constructor.cpp",
- "begin": "(\\s*+((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<14>?)+)>)\\s*)?::)*)(((?>(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))::((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))\\16((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=\\()))",
+ "begin": "(\\s*+((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<14>?)+)>)\\s*)?::)*)(((?>(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))::((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))\\16((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=\\()))",
"beginCaptures": {
"1": {
"name": "meta.head.function.definition.special.constructor.cpp"
@@ -1924,7 +1924,7 @@
{
"patterns": [
{
- "match": "(\\=)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(default)|(delete))",
+ "match": "(\\=)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(default)|(delete))",
"captures": {
"1": {
"name": "keyword.operator.assignment.cpp"
@@ -2094,7 +2094,7 @@
]
},
"control_flow_keywords": {
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\{)",
+ "begin": "(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\{)",
"beginCaptures": {
"1": {
"name": "meta.qualified_type.cpp",
@@ -2403,7 +2403,7 @@
},
"decltype": {
"contentName": "meta.arguments.decltype.cpp",
- "begin": "((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"name": "keyword.operator.functionlike.cpp keyword.other.decltype.cpp storage.type.decltype.cpp"
@@ -2451,7 +2451,7 @@
},
"decltype_specifier": {
"contentName": "meta.arguments.decltype.cpp",
- "begin": "((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"name": "keyword.operator.functionlike.cpp keyword.other.decltype.cpp storage.type.decltype.cpp"
@@ -2499,7 +2499,7 @@
},
"default_statement": {
"name": "meta.conditional.case.cpp",
- "begin": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?:constexpr|explicit|mutable|virtual|inline|friend)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*)(~(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:(?:constexpr|explicit|mutable|virtual|inline|friend)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*)(~(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(default)|(delete))",
+ "match": "(\\=)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(default)|(delete))",
"captures": {
"1": {
"name": "keyword.operator.assignment.cpp"
@@ -2773,7 +2773,7 @@
},
"destructor_root": {
"name": "meta.function.definition.special.member.destructor.cpp",
- "begin": "(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<14>?)+)>)\\s*)?::)*)(((?>(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))::((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))~\\16((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=\\()))",
+ "begin": "(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<14>?)+)>)\\s*)?::)*)(((?>(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))::((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))~\\16((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=\\()))",
"beginCaptures": {
"1": {
"name": "meta.head.function.definition.special.member.destructor.cpp"
@@ -2964,7 +2964,7 @@
{
"patterns": [
{
- "match": "(\\=)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(default)|(delete))",
+ "match": "(\\=)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(default)|(delete))",
"captures": {
"1": {
"name": "keyword.operator.assignment.cpp"
@@ -3053,7 +3053,7 @@
},
"diagnostic": {
"name": "meta.preprocessor.diagnostic.$reference(directive).cpp",
- "begin": "((?:^)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(#)\\s*((?:error|warning)))\\b\\s*",
+ "begin": "((?:^)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(#)\\s*((?:error|warning)))\\b\\s*",
"beginCaptures": {
"1": {
"name": "keyword.control.directive.diagnostic.$7.cpp"
@@ -3179,7 +3179,7 @@
},
"enum_block": {
"name": "meta.block.enum.cpp",
- "begin": "(((?(?:(?>[^<>]*)\\g<15>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<15>?)+)>)\\s*)?(::))?\\s*((?(?:(?>[^<>]*)\\g<15>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<15>?)+)>)\\s*)?(::))?\\s*((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))\\b(?!override\\W|override\\$|final\\W|final\\$)((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=\\S)(?![:{])",
+ "match": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))\\b(?!override\\W|override\\$|final\\W|final\\$)((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=\\S)(?![:{])",
"captures": {
"1": {
"name": "storage.type.enum.declare.cpp"
@@ -3347,7 +3347,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -3662,7 +3662,7 @@
]
},
"exception_keywords": {
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(extern)(?=\\s*\\\"))",
+ "begin": "(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(extern)(?=\\s*\\\"))",
"beginCaptures": {
"1": {
"name": "meta.head.extern.cpp"
@@ -3859,7 +3859,7 @@
]
},
"function_call": {
- "begin": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<12>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(((?(?:(?>[^<>]*)\\g<12>?)+)>)\\s*)?(\\()",
+ "begin": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<12>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(((?(?:(?>[^<>]*)\\g<12>?)+)>)\\s*)?(\\()",
"beginCaptures": {
"1": {
"patterns": [
@@ -3933,7 +3933,7 @@
},
"function_definition": {
"name": "meta.function.definition.cpp",
- "begin": "((?:(?:^|\\G|(?<=;|\\}))|(?<=>))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*)(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<70>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<70>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<70>?)+)>)\\s*)?(?![\\w<:.]))(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<70>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=\\())",
+ "begin": "((?:(?:^|\\G|(?<=;|\\}))|(?<=>))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?:((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*)(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<70>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<70>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<70>?)+)>)\\s*)?(?![\\w<:.]))(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<70>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=\\())",
"beginCaptures": {
"1": {
"name": "meta.head.function.definition.cpp"
@@ -3994,7 +3994,7 @@
"11": {
"patterns": [
{
- "match": "((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))",
+ "match": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))",
"captures": {
"1": {
"name": "storage.modifier.$1.cpp"
@@ -4228,7 +4228,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -4529,7 +4529,7 @@
]
},
"function_pointer": {
- "begin": "(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()(\\*)\\s*((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)?)\\s*(?:(\\[)(\\w*)(\\])\\s*)*(\\))\\s*(\\()",
+ "begin": "(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()(\\*)\\s*((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)?)\\s*(?:(\\[)(\\w*)(\\])\\s*)*(\\))\\s*(\\()",
"beginCaptures": {
"1": {
"name": "meta.qualified_type.cpp",
@@ -4703,7 +4703,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -4843,7 +4843,7 @@
"name": "punctuation.section.parameters.begin.bracket.round.function.pointer.cpp"
}
},
- "end": "(\\))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=[{=,);]|\\n)(?!\\()|(?=(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=[{=,);]|\\n)(?!\\()|(?=(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()(\\*)\\s*((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)?)\\s*(?:(\\[)(\\w*)(\\])\\s*)*(\\))\\s*(\\()",
+ "begin": "(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()(\\*)\\s*((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)?)\\s*(?:(\\[)(\\w*)(\\])\\s*)*(\\))\\s*(\\()",
"beginCaptures": {
"1": {
"name": "meta.qualified_type.cpp",
@@ -5055,7 +5055,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -5195,7 +5195,7 @@
"name": "punctuation.section.parameters.begin.bracket.round.function.pointer.cpp"
}
},
- "end": "(\\))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=[{=,);]|\\n)(?!\\()|(?=(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=[{=,);]|\\n)(?!\\()|(?=(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)",
+ "match": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)",
"captures": {
"1": {
"name": "keyword.control.goto.cpp"
@@ -5335,7 +5335,7 @@
}
},
"include": {
- "match": "(?:^)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((#)\\s*((?:include|include_next))\\b)\\s*(?:(?:(?:((<)[^>]*(>?)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:\\n|$)|(?=\\/\\/)))|((\\\")[^\\\"]*(\\\"?)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:\\n|$)|(?=\\/\\/))))|(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*(?:\\.(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)*((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:\\n|$)|(?=(?:\\/\\/|;)))))|((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:\\n|$)|(?=(?:\\/\\/|;))))",
+ "match": "(?:^)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((#)\\s*((?:include|include_next))\\b)\\s*(?:(?:(?:((<)[^>]*(>?)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:\\n|$)|(?=\\/\\/)))|((\\\")[^\\\"]*(\\\"?)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:\\n|$)|(?=\\/\\/))))|(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*(?:\\.(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)*((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:\\n|$)|(?=(?:\\/\\/|;)))))|((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:\\n|$)|(?=(?:\\/\\/|;))))",
"captures": {
"1": {
"patterns": [
@@ -5535,7 +5535,7 @@
"name": "storage.type.modifier.virtual.cpp"
},
{
- "match": "(?<=protected|virtual|private|public|,|:)\\s*(?!(?:(?:protected|private|public)|virtual))(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))",
+ "match": "(?<=protected|virtual|private|public|,|:)\\s*(?!(?:(?:protected|private|public)|virtual))(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))",
"captures": {
"1": {
"name": "meta.qualified_type.cpp",
@@ -5734,7 +5734,7 @@
"name": "invalid.illegal.unexpected.punctuation.definition.comment.end.cpp"
},
"label": {
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(:)",
+ "match": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(:)",
"captures": {
"1": {
"patterns": [
@@ -5795,7 +5795,7 @@
}
},
"lambdas": {
- "begin": "((?:(?<=[^\\s]|^)(?])|(?<=\\Wreturn|^return))\\s*(\\[(?!\\[))((?:[^\\]\\[]*\\[.*?\\](?!\\s*\\[)[^\\]\\[]*?)*[^\\]\\[]*?)(\\](?!\\[)))",
+ "begin": "((?:(?<=[^\\s]|^)(?])|(?<=\\Wreturn|^return))\\s*(\\[(?!\\[| *+\"| *+\\d))((?>(?:[^\\[\\]]|((?(?:(?>[^\\[\\]]*)\\g<4>?)+)\\]))*))(\\](?!\\[)))",
"beginCaptures": {
"2": {
"name": "punctuation.definition.capture.begin.lambda.cpp"
@@ -5807,7 +5807,7 @@
"include": "#the_this_keyword"
},
{
- "match": "((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?=\\]|\\z|$)|(,))|(\\=))",
+ "match": "((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?=\\]|\\z|$)|(,))|(\\=))",
"captures": {
"1": {
"name": "variable.parameter.capture.cpp"
@@ -5850,7 +5850,7 @@
}
]
},
- "4": {
+ "5": {
"name": "punctuation.definition.capture.end.lambda.cpp"
}
},
@@ -5919,7 +5919,7 @@
},
"line": {
"name": "meta.preprocessor.line.cpp",
- "begin": "((?:^)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(#)\\s*line\\b)",
+ "begin": "((?:^)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(#)\\s*line\\b)",
"beginCaptures": {
"1": {
"name": "keyword.control.directive.line.cpp"
@@ -5987,7 +5987,7 @@
},
"macro": {
"name": "meta.preprocessor.macro.cpp",
- "begin": "((?:^)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(#)\\s*define\\b)\\s*((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(#)\\s*define\\b)\\s*((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\*|->)))((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*\\s*(?:(?:(?:\\.\\*|\\.))|(?:(?:->\\*|->)))\\s*)*)\\s*(\\b(?!uint_least64_t[^(?-mix:\\w)]|uint_least16_t[^(?-mix:\\w)]|uint_least32_t[^(?-mix:\\w)]|int_least16_t[^(?-mix:\\w)]|uint_fast64_t[^(?-mix:\\w)]|uint_fast32_t[^(?-mix:\\w)]|uint_fast16_t[^(?-mix:\\w)]|uint_least8_t[^(?-mix:\\w)]|int_least64_t[^(?-mix:\\w)]|int_least32_t[^(?-mix:\\w)]|int_fast32_t[^(?-mix:\\w)]|int_fast16_t[^(?-mix:\\w)]|int_least8_t[^(?-mix:\\w)]|uint_fast8_t[^(?-mix:\\w)]|int_fast64_t[^(?-mix:\\w)]|int_fast8_t[^(?-mix:\\w)]|suseconds_t[^(?-mix:\\w)]|useconds_t[^(?-mix:\\w)]|in_addr_t[^(?-mix:\\w)]|uintmax_t[^(?-mix:\\w)]|uintmax_t[^(?-mix:\\w)]|uintptr_t[^(?-mix:\\w)]|blksize_t[^(?-mix:\\w)]|in_port_t[^(?-mix:\\w)]|intmax_t[^(?-mix:\\w)]|unsigned[^(?-mix:\\w)]|blkcnt_t[^(?-mix:\\w)]|uint32_t[^(?-mix:\\w)]|u_quad_t[^(?-mix:\\w)]|uint16_t[^(?-mix:\\w)]|intmax_t[^(?-mix:\\w)]|uint64_t[^(?-mix:\\w)]|intptr_t[^(?-mix:\\w)]|swblk_t[^(?-mix:\\w)]|wchar_t[^(?-mix:\\w)]|u_short[^(?-mix:\\w)]|qaddr_t[^(?-mix:\\w)]|caddr_t[^(?-mix:\\w)]|daddr_t[^(?-mix:\\w)]|fixpt_t[^(?-mix:\\w)]|nlink_t[^(?-mix:\\w)]|segsz_t[^(?-mix:\\w)]|clock_t[^(?-mix:\\w)]|ssize_t[^(?-mix:\\w)]|int16_t[^(?-mix:\\w)]|int32_t[^(?-mix:\\w)]|int64_t[^(?-mix:\\w)]|uint8_t[^(?-mix:\\w)]|int8_t[^(?-mix:\\w)]|mode_t[^(?-mix:\\w)]|quad_t[^(?-mix:\\w)]|ushort[^(?-mix:\\w)]|u_long[^(?-mix:\\w)]|u_char[^(?-mix:\\w)]|double[^(?-mix:\\w)]|size_t[^(?-mix:\\w)]|signed[^(?-mix:\\w)]|time_t[^(?-mix:\\w)]|key_t[^(?-mix:\\w)]|ino_t[^(?-mix:\\w)]|gid_t[^(?-mix:\\w)]|dev_t[^(?-mix:\\w)]|div_t[^(?-mix:\\w)]|float[^(?-mix:\\w)]|u_int[^(?-mix:\\w)]|uid_t[^(?-mix:\\w)]|short[^(?-mix:\\w)]|off_t[^(?-mix:\\w)]|pid_t[^(?-mix:\\w)]|id_t[^(?-mix:\\w)]|bool[^(?-mix:\\w)]|char[^(?-mix:\\w)]|id_t[^(?-mix:\\w)]|uint[^(?-mix:\\w)]|void[^(?-mix:\\w)]|long[^(?-mix:\\w)]|auto[^(?-mix:\\w)]|int[^(?-mix:\\w)])(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*\\b(?!\\())",
+ "match": "(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\*|->)))((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*\\s*(?:(?:(?:\\.\\*|\\.))|(?:(?:->\\*|->)))\\s*)*)\\s*(\\b(?!uint_least64_t[^(?-mix:\\w)]|uint_least16_t[^(?-mix:\\w)]|uint_least32_t[^(?-mix:\\w)]|int_least16_t[^(?-mix:\\w)]|uint_fast64_t[^(?-mix:\\w)]|uint_fast32_t[^(?-mix:\\w)]|uint_fast16_t[^(?-mix:\\w)]|uint_least8_t[^(?-mix:\\w)]|int_least64_t[^(?-mix:\\w)]|int_least32_t[^(?-mix:\\w)]|int_fast32_t[^(?-mix:\\w)]|int_fast16_t[^(?-mix:\\w)]|int_least8_t[^(?-mix:\\w)]|uint_fast8_t[^(?-mix:\\w)]|int_fast64_t[^(?-mix:\\w)]|int_fast8_t[^(?-mix:\\w)]|suseconds_t[^(?-mix:\\w)]|useconds_t[^(?-mix:\\w)]|in_addr_t[^(?-mix:\\w)]|uintmax_t[^(?-mix:\\w)]|uintmax_t[^(?-mix:\\w)]|uintptr_t[^(?-mix:\\w)]|blksize_t[^(?-mix:\\w)]|in_port_t[^(?-mix:\\w)]|intmax_t[^(?-mix:\\w)]|unsigned[^(?-mix:\\w)]|blkcnt_t[^(?-mix:\\w)]|uint32_t[^(?-mix:\\w)]|u_quad_t[^(?-mix:\\w)]|uint16_t[^(?-mix:\\w)]|intmax_t[^(?-mix:\\w)]|uint64_t[^(?-mix:\\w)]|intptr_t[^(?-mix:\\w)]|swblk_t[^(?-mix:\\w)]|wchar_t[^(?-mix:\\w)]|u_short[^(?-mix:\\w)]|qaddr_t[^(?-mix:\\w)]|caddr_t[^(?-mix:\\w)]|daddr_t[^(?-mix:\\w)]|fixpt_t[^(?-mix:\\w)]|nlink_t[^(?-mix:\\w)]|segsz_t[^(?-mix:\\w)]|clock_t[^(?-mix:\\w)]|ssize_t[^(?-mix:\\w)]|int16_t[^(?-mix:\\w)]|int32_t[^(?-mix:\\w)]|int64_t[^(?-mix:\\w)]|uint8_t[^(?-mix:\\w)]|int8_t[^(?-mix:\\w)]|mode_t[^(?-mix:\\w)]|quad_t[^(?-mix:\\w)]|ushort[^(?-mix:\\w)]|u_long[^(?-mix:\\w)]|u_char[^(?-mix:\\w)]|double[^(?-mix:\\w)]|size_t[^(?-mix:\\w)]|signed[^(?-mix:\\w)]|time_t[^(?-mix:\\w)]|key_t[^(?-mix:\\w)]|ino_t[^(?-mix:\\w)]|gid_t[^(?-mix:\\w)]|dev_t[^(?-mix:\\w)]|div_t[^(?-mix:\\w)]|float[^(?-mix:\\w)]|u_int[^(?-mix:\\w)]|uid_t[^(?-mix:\\w)]|short[^(?-mix:\\w)]|off_t[^(?-mix:\\w)]|pid_t[^(?-mix:\\w)]|id_t[^(?-mix:\\w)]|bool[^(?-mix:\\w)]|char[^(?-mix:\\w)]|id_t[^(?-mix:\\w)]|uint[^(?-mix:\\w)]|void[^(?-mix:\\w)]|long[^(?-mix:\\w)]|auto[^(?-mix:\\w)]|int[^(?-mix:\\w)])(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*\\b(?!\\())",
"captures": {
"1": {
"patterns": [
@@ -6124,7 +6124,7 @@
"9": {
"patterns": [
{
- "match": "(?<=(?:\\.\\*|\\.|->|->\\*))\\s*(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\*|->)))",
+ "match": "(?<=(?:\\.\\*|\\.|->|->\\*))\\s*(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\*|->)))",
"captures": {
"1": {
"patterns": [
@@ -6166,7 +6166,7 @@
}
},
{
- "match": "(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\*|->)))",
+ "match": "(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\*|->)))",
"captures": {
"1": {
"patterns": [
@@ -6221,7 +6221,7 @@
}
},
"memory_operators": {
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?:(delete)\\s*(\\[\\])|(delete))|(new))(?!\\w))",
+ "match": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:(?:(delete)\\s*(\\[\\])|(delete))|(new))(?!\\w))",
"captures": {
"1": {
"patterns": [
@@ -6266,7 +6266,7 @@
}
},
"method_access": {
- "begin": "(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\*|->)))((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*\\s*(?:(?:(?:\\.\\*|\\.))|(?:(?:->\\*|->)))\\s*)*)\\s*(~?(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*(\\()",
+ "begin": "(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\*|->)))((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*\\s*(?:(?:(?:\\.\\*|\\.))|(?:(?:->\\*|->)))\\s*)*)\\s*(~?(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*(\\()",
"beginCaptures": {
"1": {
"patterns": [
@@ -6308,7 +6308,7 @@
"9": {
"patterns": [
{
- "match": "(?<=(?:\\.\\*|\\.|->|->\\*))\\s*(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\*|->)))",
+ "match": "(?<=(?:\\.\\*|\\.|->|->\\*))\\s*(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\*|->)))",
"captures": {
"1": {
"patterns": [
@@ -6350,7 +6350,7 @@
}
},
{
- "match": "(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\*|->)))",
+ "match": "(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\*|->)))",
"captures": {
"1": {
"patterns": [
@@ -6423,7 +6423,7 @@
"name": "storage.modifier.$0.cpp"
},
"module_import": {
- "match": "(?:^)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((import))\\s*(?:(?:(?:((<)[^>]*(>?)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:\\n|$)|(?=\\/\\/)))|((\\\")[^\\\"]*(\\\"?)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:\\n|$)|(?=\\/\\/))))|(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*(?:\\.(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)*((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:\\n|$)|(?=(?:\\/\\/|;)))))|((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:\\n|$)|(?=(?:\\/\\/|;))))\\s*(;?)",
+ "match": "(?:^)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((import))\\s*(?:(?:(?:((<)[^>]*(>?)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:\\n|$)|(?=\\/\\/)))|((\\\")[^\\\"]*(\\\"?)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:\\n|$)|(?=\\/\\/))))|(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*(?:\\.(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)*((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:\\n|$)|(?=(?:\\/\\/|;)))))|((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:\\n|$)|(?=(?:\\/\\/|;))))\\s*(;?)",
"captures": {
"1": {
"patterns": [
@@ -6668,7 +6668,7 @@
]
},
"namespace_alias": {
- "match": "(?(?:(?>[^<>]*)\\g<9>?)+)>)\\s*)?::)*\\s*+)\\s*((?(?:(?>[^<>]*)\\g<9>?)+)>)\\s*)?::)*\\s*+)\\s*((?(?:(?>[^<>]*)\\g<5>?)+)>)\\s*)?::)*\\s*+)\\s*((?(?:(?>[^<>]*)\\g<5>?)+)>)\\s*)?::)*\\s*+)\\s*((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"name": "keyword.operator.functionlike.cpp keyword.operator.noexcept.cpp"
@@ -6848,7 +6848,7 @@
]
},
"non_primitive_types": {
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<67>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<67>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<67>?)+)>)\\s*)?(?![\\w<:.]))(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<67>?)+)>)\\s*)?::)*)(operator)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<67>?)+)>)\\s*)?::)*)(?:(?:((?:delete\\[\\]|delete|new\\[\\]|<=>|<<=|new|>>=|\\->\\*|\\/=|%=|&=|>=|\\|=|\\+\\+|\\-\\-|\\(\\)|\\[\\]|\\->|\\+\\+|<<|>>|\\-\\-|<=|\\^=|==|!=|&&|\\|\\||\\+=|\\-=|\\*=|,|\\+|\\-|!|~|\\*|&|\\*|\\/|%|\\+|\\-|<|>|&|\\^|\\||=))|((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:\\[\\])?)))|(\"\")((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=\\<|\\())",
+ "begin": "((?:(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<67>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<67>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<67>?)+)>)\\s*)?(?![\\w<:.]))(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<67>?)+)>)\\s*)?::)*)(operator)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<67>?)+)>)\\s*)?::)*)(?:(?:((?:delete\\[\\]|delete|new\\[\\]|<=>|<<=|new|>>=|\\->\\*|\\/=|%=|&=|>=|\\|=|\\+\\+|\\-\\-|\\(\\)|\\[\\]|\\->|\\+\\+|<<|>>|\\-\\-|<=|\\^=|==|!=|&&|\\|\\||\\+=|\\-=|\\*=|,|\\+|\\-|!|~|\\*|&|\\*|\\/|%|\\+|\\-|<|>|&|\\^|\\||=))|((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:\\[\\])?)))|(\"\")((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=\\<|\\())",
"beginCaptures": {
"1": {
"name": "meta.head.function.definition.special.operator-overload.cpp"
@@ -7330,7 +7330,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -7606,7 +7606,7 @@
"name": "entity.name.operator.type.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -7935,7 +7935,7 @@
},
"parameter": {
"name": "meta.parameter.cpp",
- "begin": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=\\w)",
+ "begin": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=\\w)",
"beginCaptures": {
"1": {
"patterns": [
@@ -7983,7 +7983,7 @@
"include": "#vararg_ellipses"
},
{
- "match": "((?:((?:volatile|register|restrict|static|extern|const))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))+)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=,|\\)|=)",
+ "match": "((?:((?:volatile|register|restrict|static|extern|const))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))+)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=,|\\)|=)",
"captures": {
"1": {
"patterns": [
@@ -8240,7 +8240,7 @@
"include": "#assignment_operator"
},
{
- "match": "(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=\\)|,|\\[|=|\\n)",
+ "match": "(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=\\)|,|\\[|=|\\n)",
"captures": {
"1": {
"patterns": [
@@ -8328,7 +8328,7 @@
"include": "#template_call_range"
},
{
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*)",
+ "match": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*)",
"captures": {
"0": {
"patterns": [
@@ -8337,7 +8337,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -8428,7 +8428,7 @@
]
},
"parameter_class": {
- "match": "(class)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:\\[((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))\\]((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?=,|\\)|\\n)",
+ "match": "(class)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?:(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:\\[((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))\\]((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?(?=,|\\)|\\n)",
"captures": {
"1": {
"name": "storage.type.class.parameter.cpp"
@@ -8493,7 +8493,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -8685,7 +8685,7 @@
}
},
"parameter_enum": {
- "match": "(enum)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:\\[((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))\\]((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?=,|\\)|\\n)",
+ "match": "(enum)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?:(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:\\[((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))\\]((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?(?=,|\\)|\\n)",
"captures": {
"1": {
"name": "storage.type.enum.parameter.cpp"
@@ -8750,7 +8750,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -8943,7 +8943,7 @@
},
"parameter_or_maybe_value": {
"name": "meta.parameter.cpp",
- "begin": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=\\w)",
+ "begin": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=\\w)",
"beginCaptures": {
"1": {
"patterns": [
@@ -9000,7 +9000,7 @@
"include": "#vararg_ellipses"
},
{
- "match": "((?:((?:volatile|register|restrict|static|extern|const))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))+)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=,|\\)|=)",
+ "match": "((?:((?:volatile|register|restrict|static|extern|const))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))+)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=,|\\)|=)",
"captures": {
"1": {
"patterns": [
@@ -9257,7 +9257,7 @@
]
},
{
- "match": "(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=(?:\\)|,|\\[|=|\\/\\/|(?:\\n|$)))",
+ "match": "(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=(?:\\)|,|\\[|=|\\/\\/|(?:\\n|$)))",
"captures": {
"1": {
"patterns": [
@@ -9345,7 +9345,7 @@
"include": "#template_call_range"
},
{
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*)",
+ "match": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*)",
"captures": {
"0": {
"patterns": [
@@ -9354,7 +9354,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -9448,7 +9448,7 @@
]
},
"parameter_struct": {
- "match": "(struct)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:\\[((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))\\]((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?=,|\\)|\\n)",
+ "match": "(struct)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?:(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:\\[((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))\\]((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?(?=,|\\)|\\n)",
"captures": {
"1": {
"name": "storage.type.struct.parameter.cpp"
@@ -9513,7 +9513,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -9705,7 +9705,7 @@
}
},
"parameter_union": {
- "match": "(union)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:\\[((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))\\]((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?=,|\\)|\\n)",
+ "match": "(union)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?:(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:\\[((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))\\]((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?(?=,|\\)|\\n)",
"captures": {
"1": {
"name": "storage.type.union.parameter.cpp"
@@ -9770,7 +9770,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -9989,7 +9989,7 @@
]
},
"posix_reserved_types": {
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(#)\\s*pragma\\b)",
+ "begin": "((?:^)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(#)\\s*pragma\\b)",
"beginCaptures": {
"1": {
"name": "keyword.control.directive.pragma.cpp"
@@ -10078,7 +10078,7 @@
]
},
"pragma_mark": {
- "match": "((?:^)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(#)\\s*pragma\\s+mark)\\s+(.*)",
+ "match": "((?:^)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(#)\\s*pragma\\s+mark)\\s+(.*)",
"captures": {
"1": {
"name": "keyword.control.directive.pragma.pragma-mark.cpp"
@@ -10202,7 +10202,7 @@
}
},
"preprocessor_conditional_range": {
- "begin": "((?:^)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(#)\\s*((?:(?:ifndef|ifdef)|if)))",
+ "begin": "((?:^)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(#)\\s*((?:(?:ifndef|ifdef)|if)))",
"beginCaptures": {
"1": {
"name": "keyword.control.directive.conditional.$7.cpp"
@@ -10254,7 +10254,7 @@
]
},
"preprocessor_conditional_standalone": {
- "match": "(?:^)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(#)\\s*((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(#)\\s*((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<26>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<26>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<26>?)+)>)\\s*)?(?![\\w<:.])",
+ "match": "\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<26>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<26>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<26>?)+)>)\\s*)?(?![\\w<:.])",
"captures": {
"0": {
"name": "meta.qualified_type.cpp",
@@ -10777,7 +10777,7 @@
}
},
"qualifiers_and_specifiers_post_parameters": {
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?>[^<>]*)\\g<4>?)+)>)\\s*)?::)*\\s*+",
+ "match": "(::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<4>?)+)>)\\s*)?::)*\\s*+",
"captures": {
"0": {
"patterns": [
@@ -10833,7 +10833,7 @@
}
},
"scope_resolution_function_call": {
- "match": "(::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<4>?)+)>)\\s*)?::)*\\s*+",
+ "match": "(::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<4>?)+)>)\\s*)?::)*\\s*+",
"captures": {
"0": {
"patterns": [
@@ -10856,7 +10856,7 @@
}
},
"scope_resolution_function_call_inner_generated": {
- "match": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?(::)",
+ "match": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?(::)",
"captures": {
"1": {
"patterns": [
@@ -10893,7 +10893,7 @@
}
},
"scope_resolution_function_definition": {
- "match": "(::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<4>?)+)>)\\s*)?::)*\\s*+",
+ "match": "(::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<4>?)+)>)\\s*)?::)*\\s*+",
"captures": {
"0": {
"patterns": [
@@ -10916,7 +10916,7 @@
}
},
"scope_resolution_function_definition_inner_generated": {
- "match": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?(::)",
+ "match": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?(::)",
"captures": {
"1": {
"patterns": [
@@ -10953,7 +10953,7 @@
}
},
"scope_resolution_function_definition_operator_overload": {
- "match": "(::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<4>?)+)>)\\s*)?::)*\\s*+",
+ "match": "(::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<4>?)+)>)\\s*)?::)*\\s*+",
"captures": {
"0": {
"patterns": [
@@ -10976,7 +10976,7 @@
}
},
"scope_resolution_function_definition_operator_overload_inner_generated": {
- "match": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?(::)",
+ "match": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?(::)",
"captures": {
"1": {
"patterns": [
@@ -11013,7 +11013,7 @@
}
},
"scope_resolution_inner_generated": {
- "match": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?(::)",
+ "match": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?(::)",
"captures": {
"1": {
"patterns": [
@@ -11050,7 +11050,7 @@
}
},
"scope_resolution_namespace_alias": {
- "match": "(::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<4>?)+)>)\\s*)?::)*\\s*+",
+ "match": "(::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<4>?)+)>)\\s*)?::)*\\s*+",
"captures": {
"0": {
"patterns": [
@@ -11073,7 +11073,7 @@
}
},
"scope_resolution_namespace_alias_inner_generated": {
- "match": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?(::)",
+ "match": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?(::)",
"captures": {
"1": {
"patterns": [
@@ -11110,7 +11110,7 @@
}
},
"scope_resolution_namespace_block": {
- "match": "(::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<4>?)+)>)\\s*)?::)*\\s*+",
+ "match": "(::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<4>?)+)>)\\s*)?::)*\\s*+",
"captures": {
"0": {
"patterns": [
@@ -11133,7 +11133,7 @@
}
},
"scope_resolution_namespace_block_inner_generated": {
- "match": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?(::)",
+ "match": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?(::)",
"captures": {
"1": {
"patterns": [
@@ -11170,7 +11170,7 @@
}
},
"scope_resolution_namespace_using": {
- "match": "(::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<4>?)+)>)\\s*)?::)*\\s*+",
+ "match": "(::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<4>?)+)>)\\s*)?::)*\\s*+",
"captures": {
"0": {
"patterns": [
@@ -11193,7 +11193,7 @@
}
},
"scope_resolution_namespace_using_inner_generated": {
- "match": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?(::)",
+ "match": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?(::)",
"captures": {
"1": {
"patterns": [
@@ -11230,7 +11230,7 @@
}
},
"scope_resolution_parameter": {
- "match": "(::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<4>?)+)>)\\s*)?::)*\\s*+",
+ "match": "(::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<4>?)+)>)\\s*)?::)*\\s*+",
"captures": {
"0": {
"patterns": [
@@ -11253,7 +11253,7 @@
}
},
"scope_resolution_parameter_inner_generated": {
- "match": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?(::)",
+ "match": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?(::)",
"captures": {
"1": {
"patterns": [
@@ -11290,7 +11290,7 @@
}
},
"scope_resolution_template_call": {
- "match": "(::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<4>?)+)>)\\s*)?::)*\\s*+",
+ "match": "(::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<4>?)+)>)\\s*)?::)*\\s*+",
"captures": {
"0": {
"patterns": [
@@ -11313,7 +11313,7 @@
}
},
"scope_resolution_template_call_inner_generated": {
- "match": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?(::)",
+ "match": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?(::)",
"captures": {
"1": {
"patterns": [
@@ -11350,7 +11350,7 @@
}
},
"scope_resolution_template_definition": {
- "match": "(::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<4>?)+)>)\\s*)?::)*\\s*+",
+ "match": "(::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<4>?)+)>)\\s*)?::)*\\s*+",
"captures": {
"0": {
"patterns": [
@@ -11373,7 +11373,7 @@
}
},
"scope_resolution_template_definition_inner_generated": {
- "match": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?(::)",
+ "match": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<8>?)+)>)\\s*)?(::)",
"captures": {
"1": {
"patterns": [
@@ -11414,7 +11414,7 @@
"name": "punctuation.terminator.statement.cpp"
},
"simple_type": {
- "match": "(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?",
+ "match": "(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?",
"captures": {
"1": {
"name": "meta.qualified_type.cpp",
@@ -11588,7 +11588,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -11677,7 +11677,7 @@
}
},
"single_line_macro": {
- "match": "^((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))#define.*(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))#define.*(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"name": "keyword.operator.functionlike.cpp keyword.operator.sizeof.cpp"
@@ -11766,7 +11766,7 @@
},
"sizeof_variadic_operator": {
"contentName": "meta.arguments.operator.sizeof.variadic.cpp",
- "begin": "(\\bsizeof\\.\\.\\.)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "(\\bsizeof\\.\\.\\.)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"name": "keyword.operator.functionlike.cpp keyword.operator.sizeof.variadic.cpp"
@@ -11852,7 +11852,7 @@
]
},
"static_assert": {
- "begin": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"patterns": [
@@ -11939,7 +11939,7 @@
]
},
"storage_specifiers": {
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(DLLEXPORT)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(final)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(:)((?>[^{]*)))?))",
+ "begin": "((((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(DLLEXPORT)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(final)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(:)((?>[^{]*)))?))",
"beginCaptures": {
"1": {
"name": "meta.head.struct.cpp"
@@ -12450,7 +12450,7 @@
]
},
"struct_declare": {
- "match": "(struct)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))\\b(?!override\\W|override\\$|final\\W|final\\$)((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=\\S)(?![:{])",
+ "match": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))\\b(?!override\\W|override\\$|final\\W|final\\$)((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=\\S)(?![:{])",
"captures": {
"1": {
"name": "storage.type.struct.declare.cpp"
@@ -12490,7 +12490,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -12633,7 +12633,7 @@
},
"switch_conditional_parentheses": {
"name": "meta.conditional.switch.cpp",
- "begin": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"patterns": [
@@ -12681,7 +12681,7 @@
},
"switch_statement": {
"name": "meta.block.switch.cpp",
- "begin": "(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)|((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*\\s+)+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))|((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*(\\.\\.\\.)\\s*((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*(?:(,)|(?=>|$))",
+ "match": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)|((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*\\s+)+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))|((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*(\\.\\.\\.)\\s*((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*(?:(,)|(?=>|$))",
"captures": {
"1": {
"patterns": [
@@ -13093,7 +13093,7 @@
]
},
"the_this_keyword": {
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?(?![\\w<:.]))\\s*(\\=)\\s*((?:typename)?)\\s*((?:(?:(?:(?:(?>\\s+)|(?:\\/\\*)(?:(?>(?:[^\\*]|(?>\\*+)[^\\/])*)(?:(?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?(?![\\w<:.]))|(.*(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(\\[)(\\w*)(\\])\\s*)?\\s*(?:(;)|\\n)",
+ "match": "(using)\\s*(?!namespace)(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?(?![\\w<:.]))\\s*(\\=)\\s*((?:typename)?)\\s*((?:(?:(?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)(?:(?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?(?![\\w<:.]))|(.*(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?(?:(\\[)(\\w*)(\\])\\s*)?\\s*(?:(;)|\\n)",
"captures": {
"1": {
"name": "keyword.other.using.directive.cpp"
@@ -13489,7 +13489,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -13620,7 +13620,7 @@
"name": "meta.declaration.type.alias.cpp"
},
"type_casting_operators": {
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(DLLEXPORT)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(final)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(:)((?>[^{]*)))?))",
+ "begin": "((((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(DLLEXPORT)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(final)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(:)((?>[^{]*)))?))",
"beginCaptures": {
"1": {
"name": "meta.head.class.cpp"
@@ -13950,7 +13950,7 @@
"end": "[\\s\\n]*(?=;)|(?=(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -14094,7 +14094,7 @@
"end": "(?<=;)|(?=(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()(\\*)\\s*((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)?)\\s*(?:(\\[)(\\w*)(\\])\\s*)*(\\))\\s*(\\()",
+ "begin": "(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()(\\*)\\s*((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)?)\\s*(?:(\\[)(\\w*)(\\])\\s*)*(\\))\\s*(\\()",
"beginCaptures": {
"1": {
"name": "meta.qualified_type.cpp",
@@ -14268,7 +14268,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -14408,7 +14408,7 @@
"name": "punctuation.section.parameters.begin.bracket.round.function.pointer.cpp"
}
},
- "end": "(\\))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=[{=,);]|\\n)(?!\\()|(?=(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=[{=,);]|\\n)(?!\\()|(?=(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(DLLEXPORT)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(final)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(:)((?>[^{]*)))?))",
+ "begin": "((((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(DLLEXPORT)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(final)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(:)((?>[^{]*)))?))",
"beginCaptures": {
"1": {
"name": "meta.head.struct.cpp"
@@ -14778,7 +14778,7 @@
"end": "[\\s\\n]*(?=;)|(?=(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -14923,7 +14923,7 @@
"patterns": [
{
"name": "meta.block.union.cpp",
- "begin": "((((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(DLLEXPORT)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(final)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(:)((?>[^{]*)))?))",
+ "begin": "((((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(DLLEXPORT)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(final)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(:)((?>[^{]*)))?))",
"beginCaptures": {
"1": {
"name": "meta.head.union.cpp"
@@ -15210,7 +15210,7 @@
"end": "[\\s\\n]*(?=;)|(?=(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -15346,7 +15346,7 @@
},
"typeid_operator": {
"contentName": "meta.arguments.operator.typeid.cpp",
- "begin": "((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"name": "keyword.operator.functionlike.cpp keyword.operator.typeid.cpp"
@@ -15393,7 +15393,7 @@
]
},
"typename": {
- "match": "(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|new|try|for|asm|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<36>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<36>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<36>?)+)>)\\s*)?(?![\\w<:.]))",
+ "match": "(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<36>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<36>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<36>?)+)>)\\s*)?(?![\\w<:.]))",
"captures": {
"1": {
"name": "storage.modifier.cpp"
@@ -15616,7 +15616,7 @@
}
},
"undef": {
- "match": "((?:^)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(#)\\s*undef\\b)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(#)\\s*undef\\b)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(DLLEXPORT)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(final)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(:)((?>[^{]*)))?))",
+ "begin": "((((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(DLLEXPORT)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(final)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(:)((?>[^{]*)))?))",
"beginCaptures": {
"1": {
"name": "meta.head.union.cpp"
@@ -15976,7 +15976,7 @@
]
},
"union_declare": {
- "match": "(union)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))\\b(?!override\\W|override\\$|final\\W|final\\$)((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=\\S)(?![:{])",
+ "match": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))\\b(?!override\\W|override\\$|final\\W|final\\$)((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=\\S)(?![:{])",
"captures": {
"1": {
"name": "storage.type.union.declare.cpp"
@@ -16016,7 +16016,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -16167,7 +16167,7 @@
},
"using_namespace": {
"name": "meta.using-namespace.cpp",
- "begin": "(?(?:(?>[^<>]*)\\g<7>?)+)>)\\s*)?::)*\\s*+)?((?(?:(?>[^<>]*)\\g<7>?)+)>)\\s*)?::)*\\s*+)?((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(((?:protected|private|public))\\s*(:))",
+ "match": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(((?:protected|private|public))\\s*(:))",
"captures": {
"1": {
"patterns": [
@@ -194,7 +194,7 @@
},
"alignas_operator": {
"contentName": "meta.arguments.operator.alignas.cpp",
- "begin": "((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"name": "keyword.operator.functionlike.cpp keyword.operator.alignas.cpp"
@@ -242,7 +242,7 @@
},
"alignof_operator": {
"contentName": "meta.arguments.operator.alignof.cpp",
- "begin": "((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"name": "keyword.operator.functionlike.cpp keyword.operator.alignof.cpp"
@@ -442,7 +442,7 @@
}
},
"builtin_storage_type_initilizer": {
- "begin": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"patterns": [
@@ -624,7 +624,7 @@
},
"case_statement": {
"name": "meta.conditional.case.cpp",
- "begin": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(DLLEXPORT)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(final)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(:)((?>[^{]*)))?))",
+ "begin": "((((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(DLLEXPORT)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(final)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(:)((?>[^{]*)))?))",
"beginCaptures": {
"1": {
"name": "meta.head.class.cpp"
@@ -966,7 +966,7 @@
]
},
"class_declare": {
- "match": "(class)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))\\b(?!override\\W|override\\$|final\\W|final\\$)((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=\\S)(?![:{])",
+ "match": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))\\b(?!override\\W|override\\$|final\\W|final\\$)((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=\\S)(?![:{])",
"captures": {
"1": {
"name": "storage.type.class.declare.cpp"
@@ -1006,7 +1006,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -1425,7 +1425,7 @@
},
"constructor_inline": {
"name": "meta.function.definition.special.constructor.cpp",
- "begin": "(^((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?:constexpr|explicit|mutable|virtual|inline|friend)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:(?:constexpr|explicit|mutable|virtual|inline|friend)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(default)|(delete))",
+ "match": "(\\=)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(default)|(delete))",
"captures": {
"1": {
"name": "keyword.operator.assignment.cpp"
@@ -1733,7 +1733,7 @@
},
"constructor_root": {
"name": "meta.function.definition.special.constructor.cpp",
- "begin": "(\\s*+((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<14>?)+)>)\\s*)?::)*)(((?>(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))::((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))\\16((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=\\()))",
+ "begin": "(\\s*+((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<14>?)+)>)\\s*)?::)*)(((?>(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))::((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))\\16((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=\\()))",
"beginCaptures": {
"1": {
"name": "meta.head.function.definition.special.constructor.cpp"
@@ -1924,7 +1924,7 @@
{
"patterns": [
{
- "match": "(\\=)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(default)|(delete))",
+ "match": "(\\=)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(default)|(delete))",
"captures": {
"1": {
"name": "keyword.operator.assignment.cpp"
@@ -2094,7 +2094,7 @@
]
},
"control_flow_keywords": {
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\{)",
+ "begin": "(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\{)",
"beginCaptures": {
"1": {
"name": "meta.qualified_type.cpp",
@@ -2403,7 +2403,7 @@
},
"decltype": {
"contentName": "meta.arguments.decltype.cpp",
- "begin": "((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"name": "keyword.operator.functionlike.cpp keyword.other.decltype.cpp storage.type.decltype.cpp"
@@ -2451,7 +2451,7 @@
},
"decltype_specifier": {
"contentName": "meta.arguments.decltype.cpp",
- "begin": "((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"name": "keyword.operator.functionlike.cpp keyword.other.decltype.cpp storage.type.decltype.cpp"
@@ -2499,7 +2499,7 @@
},
"default_statement": {
"name": "meta.conditional.case.cpp",
- "begin": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?:constexpr|explicit|mutable|virtual|inline|friend)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*)(~(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:(?:constexpr|explicit|mutable|virtual|inline|friend)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*)(~(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(default)|(delete))",
+ "match": "(\\=)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(default)|(delete))",
"captures": {
"1": {
"name": "keyword.operator.assignment.cpp"
@@ -2773,7 +2773,7 @@
},
"destructor_root": {
"name": "meta.function.definition.special.member.destructor.cpp",
- "begin": "(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<14>?)+)>)\\s*)?::)*)(((?>(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))::((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))~\\16((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=\\()))",
+ "begin": "(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<14>?)+)>)\\s*)?::)*)(((?>(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))::((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))~\\16((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=\\()))",
"beginCaptures": {
"1": {
"name": "meta.head.function.definition.special.member.destructor.cpp"
@@ -2964,7 +2964,7 @@
{
"patterns": [
{
- "match": "(\\=)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(default)|(delete))",
+ "match": "(\\=)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(default)|(delete))",
"captures": {
"1": {
"name": "keyword.operator.assignment.cpp"
@@ -3053,7 +3053,7 @@
},
"diagnostic": {
"name": "meta.preprocessor.diagnostic.$reference(directive).cpp",
- "begin": "((?:^)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(#)\\s*((?:error|warning)))\\b\\s*",
+ "begin": "((?:^)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(#)\\s*((?:error|warning)))\\b\\s*",
"beginCaptures": {
"1": {
"name": "keyword.control.directive.diagnostic.$7.cpp"
@@ -3307,7 +3307,7 @@
]
},
"enum_declare": {
- "match": "(enum)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))\\b(?!override\\W|override\\$|final\\W|final\\$)((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=\\S)(?![:{])",
+ "match": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))\\b(?!override\\W|override\\$|final\\W|final\\$)((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=\\S)(?![:{])",
"captures": {
"1": {
"name": "storage.type.enum.declare.cpp"
@@ -3347,7 +3347,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -3662,7 +3662,7 @@
]
},
"exception_keywords": {
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(extern)(?=\\s*\\\"))",
+ "begin": "(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(extern)(?=\\s*\\\"))",
"beginCaptures": {
"1": {
"name": "meta.head.extern.cpp"
@@ -3859,7 +3859,7 @@
]
},
"function_call": {
- "begin": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<12>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(((?(?:(?>[^<>]*)\\g<12>?)+)>)\\s*)?(\\()",
+ "begin": "((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<12>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(((?(?:(?>[^<>]*)\\g<12>?)+)>)\\s*)?(\\()",
"beginCaptures": {
"1": {
"patterns": [
@@ -3933,7 +3933,7 @@
},
"function_definition": {
"name": "meta.function.definition.cpp",
- "begin": "((?:(?:^|\\G|(?<=;|\\}))|(?<=>))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*)(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<70>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<70>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<70>?)+)>)\\s*)?(?![\\w<:.]))(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<70>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=\\())",
+ "begin": "((?:(?:^|\\G|(?<=;|\\}))|(?<=>))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?:((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*)(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<70>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<70>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<70>?)+)>)\\s*)?(?![\\w<:.]))(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<70>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=\\())",
"beginCaptures": {
"1": {
"name": "meta.head.function.definition.cpp"
@@ -3994,7 +3994,7 @@
"11": {
"patterns": [
{
- "match": "((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))",
+ "match": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))",
"captures": {
"1": {
"name": "storage.modifier.$1.cpp"
@@ -4228,7 +4228,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -4529,7 +4529,7 @@
]
},
"function_pointer": {
- "begin": "(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()(\\*)\\s*((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)?)\\s*(?:(\\[)(\\w*)(\\])\\s*)*(\\))\\s*(\\()",
+ "begin": "(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()(\\*)\\s*((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)?)\\s*(?:(\\[)(\\w*)(\\])\\s*)*(\\))\\s*(\\()",
"beginCaptures": {
"1": {
"name": "meta.qualified_type.cpp",
@@ -4703,7 +4703,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -4843,7 +4843,7 @@
"name": "punctuation.section.parameters.begin.bracket.round.function.pointer.cpp"
}
},
- "end": "(\\))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=[{=,);]|\\n)(?!\\()",
+ "end": "(\\))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=[{=,);]|\\n)(?!\\()",
"endCaptures": {
"1": {
"name": "punctuation.section.parameters.end.bracket.round.function.pointer.cpp"
@@ -4881,7 +4881,7 @@
]
},
"function_pointer_parameter": {
- "begin": "(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()(\\*)\\s*((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)?)\\s*(?:(\\[)(\\w*)(\\])\\s*)*(\\))\\s*(\\()",
+ "begin": "(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()(\\*)\\s*((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)?)\\s*(?:(\\[)(\\w*)(\\])\\s*)*(\\))\\s*(\\()",
"beginCaptures": {
"1": {
"name": "meta.qualified_type.cpp",
@@ -5055,7 +5055,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -5195,7 +5195,7 @@
"name": "punctuation.section.parameters.begin.bracket.round.function.pointer.cpp"
}
},
- "end": "(\\))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=[{=,);]|\\n)(?!\\()",
+ "end": "(\\))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=[{=,);]|\\n)(?!\\()",
"endCaptures": {
"1": {
"name": "punctuation.section.parameters.end.bracket.round.function.pointer.cpp"
@@ -5299,7 +5299,7 @@
]
},
"goto_statement": {
- "match": "((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)",
+ "match": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)",
"captures": {
"1": {
"name": "keyword.control.goto.cpp"
@@ -5335,7 +5335,7 @@
}
},
"include": {
- "match": "(?:^)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((#)\\s*((?:include|include_next))\\b)\\s*(?:(?:(?:((<)[^>]*(>?)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:\\n|$)|(?=\\/\\/)))|((\\\")[^\\\"]*(\\\"?)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:\\n|$)|(?=\\/\\/))))|(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*(?:\\.(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)*((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:\\n|$)|(?=(?:\\/\\/|;)))))|((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:\\n|$)|(?=(?:\\/\\/|;))))",
+ "match": "(?:^)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((#)\\s*((?:include|include_next))\\b)\\s*(?:(?:(?:((<)[^>]*(>?)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:\\n|$)|(?=\\/\\/)))|((\\\")[^\\\"]*(\\\"?)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:\\n|$)|(?=\\/\\/))))|(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*(?:\\.(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)*((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:\\n|$)|(?=(?:\\/\\/|;)))))|((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:\\n|$)|(?=(?:\\/\\/|;))))",
"captures": {
"1": {
"patterns": [
@@ -5535,7 +5535,7 @@
"name": "storage.type.modifier.virtual.cpp"
},
{
- "match": "(?<=protected|virtual|private|public|,|:)\\s*(?!(?:(?:protected|private|public)|virtual))(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))",
+ "match": "(?<=protected|virtual|private|public|,|:)\\s*(?!(?:(?:protected|private|public)|virtual))(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))",
"captures": {
"1": {
"name": "meta.qualified_type.cpp",
@@ -5734,7 +5734,7 @@
"name": "invalid.illegal.unexpected.punctuation.definition.comment.end.cpp"
},
"label": {
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(:)",
+ "match": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(:)",
"captures": {
"1": {
"patterns": [
@@ -5795,7 +5795,7 @@
}
},
"lambdas": {
- "begin": "((?:(?<=[^\\s]|^)(?])|(?<=\\Wreturn|^return))\\s*(\\[(?!\\[))((?:[^\\]\\[]*\\[.*?\\](?!\\s*\\[)[^\\]\\[]*?)*[^\\]\\[]*?)(\\](?!\\[)))",
+ "begin": "((?:(?<=[^\\s]|^)(?])|(?<=\\Wreturn|^return))\\s*(\\[(?!\\[| *+\"| *+\\d))((?>(?:[^\\[\\]]|((?(?:(?>[^\\[\\]]*)\\g<4>?)+)\\]))*))(\\](?!\\[)))",
"beginCaptures": {
"2": {
"name": "punctuation.definition.capture.begin.lambda.cpp"
@@ -5807,7 +5807,7 @@
"include": "#the_this_keyword"
},
{
- "match": "((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?=\\]|\\z|$)|(,))|(\\=))",
+ "match": "((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?=\\]|\\z|$)|(,))|(\\=))",
"captures": {
"1": {
"name": "variable.parameter.capture.cpp"
@@ -5850,7 +5850,7 @@
}
]
},
- "4": {
+ "5": {
"name": "punctuation.definition.capture.end.lambda.cpp"
}
},
@@ -5919,7 +5919,7 @@
},
"line": {
"name": "meta.preprocessor.line.cpp",
- "begin": "((?:^)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(#)\\s*line\\b)",
+ "begin": "((?:^)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(#)\\s*line\\b)",
"beginCaptures": {
"1": {
"name": "keyword.control.directive.line.cpp"
@@ -5987,7 +5987,7 @@
},
"macro": {
"name": "meta.preprocessor.macro.cpp",
- "begin": "((?:^)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(#)\\s*define\\b)\\s*((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(#)\\s*define\\b)\\s*((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\*|->)))((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*\\s*(?:(?:(?:\\.\\*|\\.))|(?:(?:->\\*|->)))\\s*)*)\\s*(\\b(?!uint_least64_t[^(?-mix:\\w)]|uint_least16_t[^(?-mix:\\w)]|uint_least32_t[^(?-mix:\\w)]|int_least16_t[^(?-mix:\\w)]|uint_fast64_t[^(?-mix:\\w)]|uint_fast32_t[^(?-mix:\\w)]|uint_fast16_t[^(?-mix:\\w)]|uint_least8_t[^(?-mix:\\w)]|int_least64_t[^(?-mix:\\w)]|int_least32_t[^(?-mix:\\w)]|int_fast32_t[^(?-mix:\\w)]|int_fast16_t[^(?-mix:\\w)]|int_least8_t[^(?-mix:\\w)]|uint_fast8_t[^(?-mix:\\w)]|int_fast64_t[^(?-mix:\\w)]|int_fast8_t[^(?-mix:\\w)]|suseconds_t[^(?-mix:\\w)]|useconds_t[^(?-mix:\\w)]|in_addr_t[^(?-mix:\\w)]|uintmax_t[^(?-mix:\\w)]|uintmax_t[^(?-mix:\\w)]|uintptr_t[^(?-mix:\\w)]|blksize_t[^(?-mix:\\w)]|in_port_t[^(?-mix:\\w)]|intmax_t[^(?-mix:\\w)]|unsigned[^(?-mix:\\w)]|blkcnt_t[^(?-mix:\\w)]|uint32_t[^(?-mix:\\w)]|u_quad_t[^(?-mix:\\w)]|uint16_t[^(?-mix:\\w)]|intmax_t[^(?-mix:\\w)]|uint64_t[^(?-mix:\\w)]|intptr_t[^(?-mix:\\w)]|swblk_t[^(?-mix:\\w)]|wchar_t[^(?-mix:\\w)]|u_short[^(?-mix:\\w)]|qaddr_t[^(?-mix:\\w)]|caddr_t[^(?-mix:\\w)]|daddr_t[^(?-mix:\\w)]|fixpt_t[^(?-mix:\\w)]|nlink_t[^(?-mix:\\w)]|segsz_t[^(?-mix:\\w)]|clock_t[^(?-mix:\\w)]|ssize_t[^(?-mix:\\w)]|int16_t[^(?-mix:\\w)]|int32_t[^(?-mix:\\w)]|int64_t[^(?-mix:\\w)]|uint8_t[^(?-mix:\\w)]|int8_t[^(?-mix:\\w)]|mode_t[^(?-mix:\\w)]|quad_t[^(?-mix:\\w)]|ushort[^(?-mix:\\w)]|u_long[^(?-mix:\\w)]|u_char[^(?-mix:\\w)]|double[^(?-mix:\\w)]|size_t[^(?-mix:\\w)]|signed[^(?-mix:\\w)]|time_t[^(?-mix:\\w)]|key_t[^(?-mix:\\w)]|ino_t[^(?-mix:\\w)]|gid_t[^(?-mix:\\w)]|dev_t[^(?-mix:\\w)]|div_t[^(?-mix:\\w)]|float[^(?-mix:\\w)]|u_int[^(?-mix:\\w)]|uid_t[^(?-mix:\\w)]|short[^(?-mix:\\w)]|off_t[^(?-mix:\\w)]|pid_t[^(?-mix:\\w)]|id_t[^(?-mix:\\w)]|bool[^(?-mix:\\w)]|char[^(?-mix:\\w)]|id_t[^(?-mix:\\w)]|uint[^(?-mix:\\w)]|void[^(?-mix:\\w)]|long[^(?-mix:\\w)]|auto[^(?-mix:\\w)]|int[^(?-mix:\\w)])(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*\\b(?!\\())",
+ "match": "(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\*|->)))((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*\\s*(?:(?:(?:\\.\\*|\\.))|(?:(?:->\\*|->)))\\s*)*)\\s*(\\b(?!uint_least64_t[^(?-mix:\\w)]|uint_least16_t[^(?-mix:\\w)]|uint_least32_t[^(?-mix:\\w)]|int_least16_t[^(?-mix:\\w)]|uint_fast64_t[^(?-mix:\\w)]|uint_fast32_t[^(?-mix:\\w)]|uint_fast16_t[^(?-mix:\\w)]|uint_least8_t[^(?-mix:\\w)]|int_least64_t[^(?-mix:\\w)]|int_least32_t[^(?-mix:\\w)]|int_fast32_t[^(?-mix:\\w)]|int_fast16_t[^(?-mix:\\w)]|int_least8_t[^(?-mix:\\w)]|uint_fast8_t[^(?-mix:\\w)]|int_fast64_t[^(?-mix:\\w)]|int_fast8_t[^(?-mix:\\w)]|suseconds_t[^(?-mix:\\w)]|useconds_t[^(?-mix:\\w)]|in_addr_t[^(?-mix:\\w)]|uintmax_t[^(?-mix:\\w)]|uintmax_t[^(?-mix:\\w)]|uintptr_t[^(?-mix:\\w)]|blksize_t[^(?-mix:\\w)]|in_port_t[^(?-mix:\\w)]|intmax_t[^(?-mix:\\w)]|unsigned[^(?-mix:\\w)]|blkcnt_t[^(?-mix:\\w)]|uint32_t[^(?-mix:\\w)]|u_quad_t[^(?-mix:\\w)]|uint16_t[^(?-mix:\\w)]|intmax_t[^(?-mix:\\w)]|uint64_t[^(?-mix:\\w)]|intptr_t[^(?-mix:\\w)]|swblk_t[^(?-mix:\\w)]|wchar_t[^(?-mix:\\w)]|u_short[^(?-mix:\\w)]|qaddr_t[^(?-mix:\\w)]|caddr_t[^(?-mix:\\w)]|daddr_t[^(?-mix:\\w)]|fixpt_t[^(?-mix:\\w)]|nlink_t[^(?-mix:\\w)]|segsz_t[^(?-mix:\\w)]|clock_t[^(?-mix:\\w)]|ssize_t[^(?-mix:\\w)]|int16_t[^(?-mix:\\w)]|int32_t[^(?-mix:\\w)]|int64_t[^(?-mix:\\w)]|uint8_t[^(?-mix:\\w)]|int8_t[^(?-mix:\\w)]|mode_t[^(?-mix:\\w)]|quad_t[^(?-mix:\\w)]|ushort[^(?-mix:\\w)]|u_long[^(?-mix:\\w)]|u_char[^(?-mix:\\w)]|double[^(?-mix:\\w)]|size_t[^(?-mix:\\w)]|signed[^(?-mix:\\w)]|time_t[^(?-mix:\\w)]|key_t[^(?-mix:\\w)]|ino_t[^(?-mix:\\w)]|gid_t[^(?-mix:\\w)]|dev_t[^(?-mix:\\w)]|div_t[^(?-mix:\\w)]|float[^(?-mix:\\w)]|u_int[^(?-mix:\\w)]|uid_t[^(?-mix:\\w)]|short[^(?-mix:\\w)]|off_t[^(?-mix:\\w)]|pid_t[^(?-mix:\\w)]|id_t[^(?-mix:\\w)]|bool[^(?-mix:\\w)]|char[^(?-mix:\\w)]|id_t[^(?-mix:\\w)]|uint[^(?-mix:\\w)]|void[^(?-mix:\\w)]|long[^(?-mix:\\w)]|auto[^(?-mix:\\w)]|int[^(?-mix:\\w)])(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*\\b(?!\\())",
"captures": {
"1": {
"patterns": [
@@ -6124,7 +6124,7 @@
"9": {
"patterns": [
{
- "match": "(?<=(?:\\.\\*|\\.|->|->\\*))\\s*(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\*|->)))",
+ "match": "(?<=(?:\\.\\*|\\.|->|->\\*))\\s*(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\*|->)))",
"captures": {
"1": {
"patterns": [
@@ -6166,7 +6166,7 @@
}
},
{
- "match": "(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\*|->)))",
+ "match": "(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\*|->)))",
"captures": {
"1": {
"patterns": [
@@ -6221,7 +6221,7 @@
}
},
"memory_operators": {
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?:(delete)\\s*(\\[\\])|(delete))|(new))(?!\\w))",
+ "match": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:(?:(delete)\\s*(\\[\\])|(delete))|(new))(?!\\w))",
"captures": {
"1": {
"patterns": [
@@ -6266,7 +6266,7 @@
}
},
"method_access": {
- "begin": "(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\*|->)))((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*\\s*(?:(?:(?:\\.\\*|\\.))|(?:(?:->\\*|->)))\\s*)*)\\s*(~?(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*(\\()",
+ "begin": "(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\*|->)))((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*\\s*(?:(?:(?:\\.\\*|\\.))|(?:(?:->\\*|->)))\\s*)*)\\s*(~?(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*(\\()",
"beginCaptures": {
"1": {
"patterns": [
@@ -6308,7 +6308,7 @@
"9": {
"patterns": [
{
- "match": "(?<=(?:\\.\\*|\\.|->|->\\*))\\s*(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\*|->)))",
+ "match": "(?<=(?:\\.\\*|\\.|->|->\\*))\\s*(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\*|->)))",
"captures": {
"1": {
"patterns": [
@@ -6350,7 +6350,7 @@
}
},
{
- "match": "(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\*|->)))",
+ "match": "(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\*|->)))",
"captures": {
"1": {
"patterns": [
@@ -6423,7 +6423,7 @@
"name": "storage.modifier.$0.cpp"
},
"module_import": {
- "match": "(?:^)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((import))\\s*(?:(?:(?:((<)[^>]*(>?)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:\\n|$)|(?=\\/\\/)))|((\\\")[^\\\"]*(\\\"?)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:\\n|$)|(?=\\/\\/))))|(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*(?:\\.(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)*((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:\\n|$)|(?=(?:\\/\\/|;)))))|((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:\\n|$)|(?=(?:\\/\\/|;))))\\s*(;?)",
+ "match": "(?:^)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((import))\\s*(?:(?:(?:((<)[^>]*(>?)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:\\n|$)|(?=\\/\\/)))|((\\\")[^\\\"]*(\\\"?)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:\\n|$)|(?=\\/\\/))))|(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*(?:\\.(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)*((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:\\n|$)|(?=(?:\\/\\/|;)))))|((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:\\n|$)|(?=(?:\\/\\/|;))))\\s*(;?)",
"captures": {
"1": {
"patterns": [
@@ -6801,7 +6801,7 @@
},
"noexcept_operator": {
"contentName": "meta.arguments.operator.noexcept.cpp",
- "begin": "((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"name": "keyword.operator.functionlike.cpp keyword.operator.noexcept.cpp"
@@ -6848,7 +6848,7 @@
]
},
"non_primitive_types": {
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<67>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<67>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<67>?)+)>)\\s*)?(?![\\w<:.]))(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<67>?)+)>)\\s*)?::)*)(operator)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<67>?)+)>)\\s*)?::)*)(?:(?:((?:delete\\[\\]|delete|new\\[\\]|<=>|<<=|new|>>=|\\->\\*|\\/=|%=|&=|>=|\\|=|\\+\\+|\\-\\-|\\(\\)|\\[\\]|\\->|\\+\\+|<<|>>|\\-\\-|<=|\\^=|==|!=|&&|\\|\\||\\+=|\\-=|\\*=|,|\\+|\\-|!|~|\\*|&|\\*|\\/|%|\\+|\\-|<|>|&|\\^|\\||=))|((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:\\[\\])?)))|(\"\")((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=\\<|\\())",
+ "begin": "((?:(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<67>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<67>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<67>?)+)>)\\s*)?(?![\\w<:.]))(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:__cdecl|__clrcall|__stdcall|__fastcall|__thiscall|__vectorcall)?)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<67>?)+)>)\\s*)?::)*)(operator)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<67>?)+)>)\\s*)?::)*)(?:(?:((?:delete\\[\\]|delete|new\\[\\]|<=>|<<=|new|>>=|\\->\\*|\\/=|%=|&=|>=|\\|=|\\+\\+|\\-\\-|\\(\\)|\\[\\]|\\->|\\+\\+|<<|>>|\\-\\-|<=|\\^=|==|!=|&&|\\|\\||\\+=|\\-=|\\*=|,|\\+|\\-|!|~|\\*|&|\\*|\\/|%|\\+|\\-|<|>|&|\\^|\\||=))|((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:\\[\\])?)))|(\"\")((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=\\<|\\())",
"beginCaptures": {
"1": {
"name": "meta.head.function.definition.special.operator-overload.cpp"
@@ -7330,7 +7330,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -7606,7 +7606,7 @@
"name": "entity.name.operator.type.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -7935,7 +7935,7 @@
},
"parameter": {
"name": "meta.parameter.cpp",
- "begin": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=\\w)",
+ "begin": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=\\w)",
"beginCaptures": {
"1": {
"patterns": [
@@ -7983,7 +7983,7 @@
"include": "#vararg_ellipses"
},
{
- "match": "((?:((?:volatile|register|restrict|static|extern|const))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))+)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=,|\\)|=)",
+ "match": "((?:((?:volatile|register|restrict|static|extern|const))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))+)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=,|\\)|=)",
"captures": {
"1": {
"patterns": [
@@ -8240,7 +8240,7 @@
"include": "#assignment_operator"
},
{
- "match": "(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=\\)|,|\\[|=|\\n)",
+ "match": "(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=\\)|,|\\[|=|\\n)",
"captures": {
"1": {
"patterns": [
@@ -8328,7 +8328,7 @@
"include": "#template_call_range"
},
{
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*)",
+ "match": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*)",
"captures": {
"0": {
"patterns": [
@@ -8337,7 +8337,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -8428,7 +8428,7 @@
]
},
"parameter_class": {
- "match": "(class)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:\\[((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))\\]((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?=,|\\)|\\n)",
+ "match": "(class)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?:(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:\\[((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))\\]((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?(?=,|\\)|\\n)",
"captures": {
"1": {
"name": "storage.type.class.parameter.cpp"
@@ -8493,7 +8493,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -8685,7 +8685,7 @@
}
},
"parameter_enum": {
- "match": "(enum)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:\\[((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))\\]((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?=,|\\)|\\n)",
+ "match": "(enum)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?:(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:\\[((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))\\]((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?(?=,|\\)|\\n)",
"captures": {
"1": {
"name": "storage.type.enum.parameter.cpp"
@@ -8750,7 +8750,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -8943,7 +8943,7 @@
},
"parameter_or_maybe_value": {
"name": "meta.parameter.cpp",
- "begin": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=\\w)",
+ "begin": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=\\w)",
"beginCaptures": {
"1": {
"patterns": [
@@ -9000,7 +9000,7 @@
"include": "#vararg_ellipses"
},
{
- "match": "((?:((?:volatile|register|restrict|static|extern|const))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))+)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=,|\\)|=)",
+ "match": "((?:((?:volatile|register|restrict|static|extern|const))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))+)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=,|\\)|=)",
"captures": {
"1": {
"patterns": [
@@ -9257,7 +9257,7 @@
]
},
{
- "match": "(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=(?:\\)|,|\\[|=|\\/\\/|(?:\\n|$)))",
+ "match": "(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=(?:\\)|,|\\[|=|\\/\\/|(?:\\n|$)))",
"captures": {
"1": {
"patterns": [
@@ -9345,7 +9345,7 @@
"include": "#template_call_range"
},
{
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*)",
+ "match": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*)",
"captures": {
"0": {
"patterns": [
@@ -9354,7 +9354,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -9448,7 +9448,7 @@
]
},
"parameter_struct": {
- "match": "(struct)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:\\[((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))\\]((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?=,|\\)|\\n)",
+ "match": "(struct)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?:(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:\\[((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))\\]((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?(?=,|\\)|\\n)",
"captures": {
"1": {
"name": "storage.type.struct.parameter.cpp"
@@ -9513,7 +9513,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -9705,7 +9705,7 @@
}
},
"parameter_union": {
- "match": "(union)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:\\[((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))\\]((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?=,|\\)|\\n)",
+ "match": "(union)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?:(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:\\[((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))\\]((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?(?=,|\\)|\\n)",
"captures": {
"1": {
"name": "storage.type.union.parameter.cpp"
@@ -9770,7 +9770,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -9989,7 +9989,7 @@
]
},
"posix_reserved_types": {
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(#)\\s*pragma\\b)",
+ "begin": "((?:^)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(#)\\s*pragma\\b)",
"beginCaptures": {
"1": {
"name": "keyword.control.directive.pragma.cpp"
@@ -10078,7 +10078,7 @@
]
},
"pragma_mark": {
- "match": "((?:^)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(#)\\s*pragma\\s+mark)\\s+(.*)",
+ "match": "((?:^)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(#)\\s*pragma\\s+mark)\\s+(.*)",
"captures": {
"1": {
"name": "keyword.control.directive.pragma.pragma-mark.cpp"
@@ -10202,7 +10202,7 @@
}
},
"preprocessor_conditional_range": {
- "begin": "((?:^)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(#)\\s*((?:(?:ifndef|ifdef)|if)))",
+ "begin": "((?:^)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(#)\\s*((?:(?:ifndef|ifdef)|if)))",
"beginCaptures": {
"1": {
"name": "keyword.control.directive.conditional.$7.cpp"
@@ -10254,7 +10254,7 @@
]
},
"preprocessor_conditional_standalone": {
- "match": "(?:^)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(#)\\s*((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(#)\\s*((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<26>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<26>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<26>?)+)>)\\s*)?(?![\\w<:.])",
+ "match": "\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<26>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<26>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<26>?)+)>)\\s*)?(?![\\w<:.])",
"captures": {
"0": {
"name": "meta.qualified_type.cpp",
@@ -10777,7 +10777,7 @@
}
},
"qualifiers_and_specifiers_post_parameters": {
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?",
+ "match": "(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?",
"captures": {
"1": {
"name": "meta.qualified_type.cpp",
@@ -11588,7 +11588,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -11677,7 +11677,7 @@
}
},
"single_line_macro": {
- "match": "^((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))#define.*(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))#define.*(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"name": "keyword.operator.functionlike.cpp keyword.operator.sizeof.cpp"
@@ -11766,7 +11766,7 @@
},
"sizeof_variadic_operator": {
"contentName": "meta.arguments.operator.sizeof.variadic.cpp",
- "begin": "(\\bsizeof\\.\\.\\.)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "(\\bsizeof\\.\\.\\.)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"name": "keyword.operator.functionlike.cpp keyword.operator.sizeof.variadic.cpp"
@@ -11852,7 +11852,7 @@
]
},
"static_assert": {
- "begin": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"patterns": [
@@ -11939,7 +11939,7 @@
]
},
"storage_specifiers": {
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(DLLEXPORT)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(final)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(:)((?>[^{]*)))?))",
+ "begin": "((((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(DLLEXPORT)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(final)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(:)((?>[^{]*)))?))",
"beginCaptures": {
"1": {
"name": "meta.head.struct.cpp"
@@ -12450,7 +12450,7 @@
]
},
"struct_declare": {
- "match": "(struct)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))\\b(?!override\\W|override\\$|final\\W|final\\$)((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=\\S)(?![:{])",
+ "match": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))\\b(?!override\\W|override\\$|final\\W|final\\$)((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=\\S)(?![:{])",
"captures": {
"1": {
"name": "storage.type.struct.declare.cpp"
@@ -12490,7 +12490,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -12633,7 +12633,7 @@
},
"switch_conditional_parentheses": {
"name": "meta.conditional.switch.cpp",
- "begin": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"patterns": [
@@ -12681,7 +12681,7 @@
},
"switch_statement": {
"name": "meta.block.switch.cpp",
- "begin": "(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)|((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*\\s+)+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))|((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*(\\.\\.\\.)\\s*((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*(?:(,)|(?=>|$))",
+ "match": "((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)|((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*\\s+)+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))|((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*(\\.\\.\\.)\\s*((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*(?:(,)|(?=>|$))",
"captures": {
"1": {
"patterns": [
@@ -13093,7 +13093,7 @@
]
},
"the_this_keyword": {
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?(?![\\w<:.]))\\s*(\\=)\\s*((?:typename)?)\\s*((?:(?:(?:(?:(?>\\s+)|(?:\\/\\*)(?:(?>(?:[^\\*]|(?>\\*+)[^\\/])*)(?:(?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?(?![\\w<:.]))|(.*(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(\\[)(\\w*)(\\])\\s*)?\\s*(?:(;)|\\n)",
+ "match": "(using)\\s*(?!namespace)(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?(?![\\w<:.]))\\s*(\\=)\\s*((?:typename)?)\\s*((?:(?:(?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)(?:(?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<58>?)+)>)\\s*)?(?![\\w<:.]))|(.*(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?(?:(\\[)(\\w*)(\\])\\s*)?\\s*(?:(;)|\\n)",
"captures": {
"1": {
"name": "keyword.other.using.directive.cpp"
@@ -13489,7 +13489,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -13620,7 +13620,7 @@
"name": "meta.declaration.type.alias.cpp"
},
"type_casting_operators": {
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(DLLEXPORT)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(final)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(:)((?>[^{]*)))?))",
+ "begin": "((((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(DLLEXPORT)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(final)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(:)((?>[^{]*)))?))",
"beginCaptures": {
"1": {
"name": "meta.head.class.cpp"
@@ -13950,7 +13950,7 @@
"end": "[\\s\\n]*(?=;)",
"patterns": [
{
- "match": "(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -14094,7 +14094,7 @@
"end": "(?<=;)",
"patterns": [
{
- "begin": "(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()(\\*)\\s*((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)?)\\s*(?:(\\[)(\\w*)(\\])\\s*)*(\\))\\s*(\\()",
+ "begin": "(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<27>?)+)>)\\s*)?(?![\\w<:.]))(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()(\\*)\\s*((?:(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)?)\\s*(?:(\\[)(\\w*)(\\])\\s*)*(\\))\\s*(\\()",
"beginCaptures": {
"1": {
"name": "meta.qualified_type.cpp",
@@ -14268,7 +14268,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -14408,7 +14408,7 @@
"name": "punctuation.section.parameters.begin.bracket.round.function.pointer.cpp"
}
},
- "end": "(\\))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=[{=,);]|\\n)(?!\\()",
+ "end": "(\\))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=[{=,);]|\\n)(?!\\()",
"endCaptures": {
"1": {
"name": "punctuation.section.parameters.end.bracket.round.function.pointer.cpp"
@@ -14448,7 +14448,7 @@
]
},
"typedef_keyword": {
- "match": "((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(DLLEXPORT)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(final)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(:)((?>[^{]*)))?))",
+ "begin": "((((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(DLLEXPORT)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(final)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(:)((?>[^{]*)))?))",
"beginCaptures": {
"1": {
"name": "meta.head.struct.cpp"
@@ -14778,7 +14778,7 @@
"end": "[\\s\\n]*(?=;)",
"patterns": [
{
- "match": "(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -14923,7 +14923,7 @@
"patterns": [
{
"name": "meta.block.union.cpp",
- "begin": "((((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(DLLEXPORT)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(final)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(:)((?>[^{]*)))?))",
+ "begin": "((((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(DLLEXPORT)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(final)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(:)((?>[^{]*)))?))",
"beginCaptures": {
"1": {
"name": "meta.head.union.cpp"
@@ -15210,7 +15210,7 @@
"end": "[\\s\\n]*(?=;)",
"patterns": [
{
- "match": "(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -15346,7 +15346,7 @@
},
"typeid_operator": {
"contentName": "meta.arguments.operator.typeid.cpp",
- "begin": "((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\()",
+ "begin": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\()",
"beginCaptures": {
"1": {
"name": "keyword.operator.functionlike.cpp keyword.operator.typeid.cpp"
@@ -15393,7 +15393,7 @@
]
},
"typename": {
- "match": "(((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<36>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<36>?)+)>)\\s*)?(::))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<36>?)+)>)\\s*)?(?![\\w<:.]))",
+ "match": "(((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(\\s*+((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?:(?:(?:unsigned|signed|short|long)|(?:struct|class|union|enum))((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(((::)?(?:((?-mix:(?!\\b(?:__has_cpp_attribute|reinterpret_cast|atomic_noexcept|atomic_cancel|atomic_commit|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|consteval|constexpr|protected|namespace|co_return|constexpr|constexpr|continue|explicit|volatile|noexcept|co_yield|noexcept|noexcept|requires|typename|decltype|operator|co_await|template|volatile|register|restrict|reflexpr|alignof|private|default|mutable|include|concept|__asm__|defined|_Pragma|alignas|typedef|warning|virtual|mutable|struct|sizeof|delete|not_eq|bitand|and_eq|xor_eq|typeid|switch|return|static|extern|inline|friend|public|ifndef|define|pragma|export|import|module|catch|throw|const|or_eq|while|compl|bitor|const|union|ifdef|class|undef|error|break|using|endif|goto|line|enum|this|case|else|elif|else|asm|try|for|new|and|xor|not|do|or|if|if)\\b)(?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*))\\s*+(((?(?:(?>[^<>]*)\\g<36>?)+)>)\\s*)?::)*\\s*+)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\s*+(((?(?:(?>[^<>]*)\\g<36>?)+)>)\\s*)?(::))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?!(?:transaction_safe_dynamic|__has_cpp_attribute|transaction_safe|reinterpret_cast|atomic_noexcept|atomic_commit|atomic_cancel|__has_include|dynamic_cast|thread_local|synchronized|static_cast|const_cast|constexpr|consteval|protected|constexpr|co_return|namespace|constexpr|noexcept|typename|decltype|template|operator|noexcept|co_yield|co_await|continue|volatile|register|restrict|explicit|override|volatile|reflexpr|noexcept|requires|default|typedef|nullptr|alignof|mutable|concept|virtual|defined|__asm__|include|_Pragma|mutable|warning|private|alignas|module|switch|not_eq|bitand|and_eq|ifndef|return|sizeof|xor_eq|export|static|delete|public|define|extern|inline|import|pragma|friend|typeid|const|compl|bitor|throw|or_eq|while|catch|break|false|final|const|endif|ifdef|undef|error|using|audit|axiom|line|else|elif|true|NULL|case|goto|else|this|new|asm|not|and|xor|try|for|if|do|or|if)\\b)((?:[a-zA-Z_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))(?:[a-zA-Z0-9_]|(?:\\\\u[0-9a-fA-F]{4}|\\\\U[0-9a-fA-F]{8}))*)\\b(((?(?:(?>[^<>]*)\\g<36>?)+)>)\\s*)?(?![\\w<:.]))",
"captures": {
"1": {
"name": "storage.modifier.cpp"
@@ -15616,7 +15616,7 @@
}
},
"undef": {
- "match": "((?:^)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(#)\\s*undef\\b)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(#)\\s*undef\\b)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(DLLEXPORT)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?:(?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(final)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(:)((?>[^{]*)))?))",
+ "begin": "((((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))|((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\))))|(?={))(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(DLLEXPORT)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?((?:(?:(?:\\[\\[.*?\\]\\]|__attribute(?:__)?\\(\\(.*?\\)\\))|__declspec\\(.*?\\))|alignas\\(.*?\\))(?!\\)))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?:(?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(final)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))?(?:((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(:)((?>[^{]*)))?))",
"beginCaptures": {
"1": {
"name": "meta.head.union.cpp"
@@ -15976,7 +15976,7 @@
]
},
"union_declare": {
- "match": "(union)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))?(?:(?:\\&|\\*)((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))*(?:\\&|\\*))?((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))\\b(?!override\\W|override\\$|final\\W|final\\$)((?\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))(?=\\S)(?![:{])",
+ "match": "((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))?(?:(?:\\&|\\*)((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))))*(?:\\&|\\*))?((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))\\b(?!override\\W|override\\$|final\\W|final\\$)((?(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))(?=\\S)(?![:{])",
"captures": {
"1": {
"name": "storage.type.union.declare.cpp"
@@ -16016,7 +16016,7 @@
"name": "storage.modifier.pointer.cpp"
},
{
- "match": "(?:\\&((?:(?:(?>\\s+)|(\\/\\*)((?>(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+?|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z)))){2,}\\&",
+ "match": "(?:\\&((?>(?:(?:(?>(?(?:[^\\*]|(?>\\*+)[^\\/])*)((?>\\*+)\\/)))+|(?:(?:(?:(?:\\b|(?<=\\W))|(?=\\W))|\\A)|\\Z))))){2,}\\&",
"captures": {
"1": {
"patterns": [
@@ -16217,4 +16217,4 @@
]
}
}
-}
\ No newline at end of file
+}
diff --git a/extensions/cpp/syntaxes/platform.tmLanguage.json b/extensions/cpp/syntaxes/platform.tmLanguage.json
index 14fb45016c0e5..0147e6629a2b0 100644
--- a/extensions/cpp/syntaxes/platform.tmLanguage.json
+++ b/extensions/cpp/syntaxes/platform.tmLanguage.json
@@ -4,23 +4,131 @@
"If you want to provide a fix or improvement, please create a pull request against the original repository.",
"Once accepted there, we are happy to receive an update request."
],
- "version": "https://github.com/textmate/c.tmbundle/commit/9aa365882274ca52f01722f3dbb169b9539a20ee",
+ "version": "https://github.com/textmate/c.tmbundle/commit/60daf83b9d45329524f7847a75e9298b3aae5805",
"name": "Platform",
"scopeName": "source.c.platform",
- "comment": "This file was generated with clang-C using MacOSX10.12.sdk",
+ "comment": "This file was generated with clang-C using MacOSX10.15.sdk",
"patterns": [
+ {
+ "match": "\\bkAudioUnitSubType_3DMixer\\b",
+ "name": "invalid.deprecated.10.10.support.constant.c"
+ },
{
"match": "\\bkCF(?:CalendarUnitWeek|Gregorian(?:AllUnits|Units(?:Days|Hours|M(?:inutes|onths)|Seconds|Years)))\\b",
"name": "invalid.deprecated.10.10.support.constant.cf.c"
},
+ {
+ "match": "\\bLS(?:ApplicationParameters|LaunchFSRefSpec)\\b",
+ "name": "invalid.deprecated.10.10.support.type.c"
+ },
{
"match": "\\bCFGregorian(?:Date|Units)\\b",
"name": "invalid.deprecated.10.10.support.type.cf.c"
},
{
- "match": "\\bkCFURL(?:CustomIconKey|EffectiveIconKey|LabelColorKey)\\b",
+ "match": "\\bkLSItem(?:ContentType|Display(?:Kind|Name)|Extension(?:IsHidden)?|File(?:Creator|Type)|IsInvisible|QuarantineProperties|RoleHandlerDisplayName)\\b",
+ "name": "invalid.deprecated.10.10.support.variable.c"
+ },
+ {
+ "match": "\\bk(?:AudioUnitProperty_(?:3DMixer(?:AttenuationCurve|Distance(?:Atten|Params)|RenderingFlags)|D(?:istanceAttenuationData|opplerShift)|ReverbPreset)|CT(?:Adobe(?:CNS1CharacterCollection|GB1CharacterCollection|Japan(?:1CharacterCollection|2CharacterCollection)|Korea1CharacterCollection)|CenterTextAlignment|Font(?:A(?:lertHeaderFontType|pplicationFontType)|ControlContentFontType|DefaultOrientation|EmphasizedSystem(?:DetailFontType|FontType)|HorizontalOrientation|LabelFontType|M(?:e(?:nu(?:Item(?:CmdKeyFontType|FontType|MarkFontType)|TitleFontType)|ssageFontType)|ini(?:EmphasizedSystemFontType|SystemFontType))|NoFontType|P(?:aletteFontType|ushButtonFontType)|S(?:mall(?:EmphasizedSystemFontType|SystemFontType|ToolbarFontType)|ystem(?:DetailFontType|FontType))|Tool(?:TipFontType|barFontType)|U(?:serF(?:ixedPitchFontType|ontType)|tilityWindowTitleFontType)|V(?:erticalOrientation|iewsFontType)|WindowTitleFontType)|IdentityMappingCharacterCollection|JustifiedTextAlignment|LeftTextAlignment|NaturalTextAlignment|RightTextAlignment)|LS(?:HandlerOptions(?:Default|IgnoreCreator)|ItemInfo(?:App(?:IsScriptable|Prefers(?:Classic|Native))|ExtensionIsHidden|Is(?:A(?:liasFile|pplication)|C(?:lassicApp|ontainer)|Invisible|NativeApp|P(?:ackage|lainFile)|Symlink|Volume))|Launch(?:InClassic|StartClassic)|Request(?:A(?:ll(?:Flags|Info)|ppTypeFlags)|BasicFlagsOnly|Extension(?:FlagsOnly)?|IconAndKind|TypeCreator)))\\b",
+ "name": "invalid.deprecated.10.11.support.constant.c"
+ },
+ {
+ "match": "\\b(?:AUDistanceAttenuationData|LSItemInfoRecord)\\b",
+ "name": "invalid.deprecated.10.11.support.type.c"
+ },
+ {
+ "match": "\\bk(?:C(?:F(?:FTPResource(?:Group|Link|Mod(?:Date|e)|Name|Owner|Size|Type)|Stream(?:NetworkServiceTypeVoIP|Property(?:FTP(?:AttemptPersistentConnection|F(?:etchResourceInfo|ileTransferOffset)|P(?:assword|roxy(?:Host|P(?:assword|ort)|User)?)|ResourceSize|Use(?:PassiveMode|rName))|HTTP(?:AttemptPersistentConnection|Final(?:Request|URL)|Proxy(?:Host|Port)?|Re(?:questBytesWrittenCount|sponseHeader)|S(?:Proxy(?:Host|Port)|houldAutoredirect)))))|GImagePropertyExifSubsecTimeOrginal|TCharacterShapeAttributeName)|IOSurfaceIsGlobal|LSSharedFileList(?:Favorite(?:Items|Volumes)|Item(?:BeforeFirst|Hidden|Last)|LoginItemHidden|Recent(?:ApplicationItems|DocumentItems|ItemsMaxAmount|ServerItems)|SessionLoginItems|Volumes(?:ComputerVisible|NetworkVisible))|SecUseNoAuthenticationUI)\\b",
+ "name": "invalid.deprecated.10.11.support.variable.c"
+ },
+ {
+ "match": "\\bkLSLaunch(?:HasUntrustedContents|InhibitBGOnly|NoParams)\\b",
+ "name": "invalid.deprecated.10.12.support.constant.c"
+ },
+ {
+ "match": "\\bOSSpinLock\\b",
+ "name": "invalid.deprecated.10.12.support.type.c"
+ },
+ {
+ "match": "\\bkSC(?:EntNetPPTP|NetworkInterfaceTypePPTP|ValNetInterfaceSubTypePPTP)\\b",
+ "name": "invalid.deprecated.10.12.support.variable.c"
+ },
+ {
+ "match": "\\bkCF(?:StreamSocketSecurityLevelSSLv(?:2|3)|URL(?:CustomIconKey|EffectiveIconKey|LabelColorKey))\\b",
"name": "invalid.deprecated.10.12.support.variable.cf.c"
},
+ {
+ "match": "\\bk(?:C(?:FNetDiagnostic(?:Connection(?:Down|Indeterminate|Up)|Err|NoErr)|TFontManagerAutoActivationPromptUser)|SecAccessControlTouchID(?:Any|CurrentSet))\\b",
+ "name": "invalid.deprecated.10.13.support.constant.c"
+ },
+ {
+ "match": "\\bCFNetDiagnosticStatus\\b",
+ "name": "invalid.deprecated.10.13.support.type.c"
+ },
+ {
+ "match": "\\bkS(?:CPropNetInterfaceSupportsModemOnHold|SLSessionConfig_(?:3DES_fallback|RC4_fallback|TLSv1_(?:3DES_fallback|RC4_fallback)|default)|ecTrustCertificateTransparencyWhiteList)\\b",
+ "name": "invalid.deprecated.10.13.support.variable.c"
+ },
+ {
+ "match": "\\bk(?:CVOpenGL(?:Buffer(?:Height|InternalFormat|MaximumMipmapLevel|PoolM(?:aximumBufferAgeKey|inimumBufferCountKey)|Target|Width)|TextureCacheChromaSamplingMode(?:Automatic|BestPerformance|HighestQuality|Key))|SecAttrAccessibleAlways(?:ThisDeviceOnly)?)\\b",
+ "name": "invalid.deprecated.10.14.support.variable.c"
+ },
+ {
+ "match": "\\bk(?:DTLSProtocol1(?:2)?|S(?:SL(?:Aborted|C(?:l(?:ient(?:Cert(?:None|Re(?:jected|quested)|Sent)|Side)|osed)|onnected)|DatagramType|Handshake|Idle|Protocol(?:2|3(?:Only)?|All|Unknown)|S(?:e(?:rverSide|ssionOption(?:Allow(?:Renegotiation|ServerIdentityChange)|BreakOn(?:C(?:ertRequested|lient(?:Auth|Hello))|ServerAuth)|EnableSessionTickets|Fal(?:lback|seStart)|SendOneByteRecord))|treamType))|ecDataAccessEvent(?:Mask)?)|TLSProtocol(?:1(?:1|2|3|Only)?|MaxSupported))\\b",
+ "name": "invalid.deprecated.10.15.support.constant.c"
+ },
+ {
+ "match": "\\bk(?:MIDIPropertyNameConfiguration|S(?:CPropNetPPP(?:AuthEAPPlugins|Plugins)|SLSessionConfig_(?:ATSv1(?:_noPFS)?|TLSv1_fallback|anonymous|legacy(?:_DHE)?|standard)))\\b",
+ "name": "invalid.deprecated.10.15.support.variable.c"
+ },
+ {
+ "match": "\\bkCGColorSpace(?:DisplayP3_PQ_EOTF|ITUR_2020_PQ_EOTF)\\b",
+ "name": "invalid.deprecated.10.16.support.variable.quartz.c"
+ },
+ {
+ "match": "\\bkMIDIProperty(?:FactoryPatchNameFile|UserPatchNameFile)\\b",
+ "name": "invalid.deprecated.10.2.support.variable.c"
+ },
+ {
+ "match": "\\bkCFNetServiceFlagIsRegistrationDomain\\b",
+ "name": "invalid.deprecated.10.4.support.constant.c"
+ },
+ {
+ "match": "\\bk(?:MDItemFS(?:Exists|Is(?:Readable|Writeable))|S(?:CPropUsersConsoleUser(?:GID|Name|UID)|KLanguageTypes))\\b",
+ "name": "invalid.deprecated.10.4.support.variable.c"
+ },
+ {
+ "match": "\\bkMDItemSupportFileType\\b",
+ "name": "invalid.deprecated.10.5.support.variable.c"
+ },
+ {
+ "match": "\\b(?:CM(?:2(?:Header|ProfileHandle)|4Header|A(?:daptationMatrixType|ppleProfileHeader)|B(?:itmap(?:C(?:allBack(?:ProcPtr|UPP)|olorSpace))?|ufferLocation)|C(?:MY(?:Color|KColor)|hromaticAdaptation|o(?:lor|ncat(?:CallBack(?:ProcPtr|UPP)|ProfileSet))|urveType)|D(?:at(?:aType|eTime(?:Type)?)|evice(?:I(?:D|nfoPtr)|Profile(?:ArrayPtr|I(?:D|nfo)|Scope)|State)|isplayIDType)|F(?:ixedXY(?:Color|ZColor)|loatBitmap)|GrayColor|H(?:LSColor|SVColor|andleLocation)|I(?:ntentCRDVMSize|terateDevice(?:InfoProcPtr|ProfileProcPtr))|L(?:ab(?:Color|ToLabProcPtr)|u(?:t(?:16Type|8Type)|vColor))|M(?:I(?:nfo|terate(?:ProcPtr|UPP))|akeAndModel(?:Type)?|easurementType|ulti(?:Funct(?:CLUTType|LutB2AType)|LocalizedUniCode(?:EntryRec|Type)|channel(?:5Color|6Color|7Color|8Color)))|Na(?:medColor(?:2(?:EntryType|Type)|Type)?|tiveDisplayInfo(?:Type)?)|P(?:S2CRDVMSizeType|a(?:rametricCurveType|thLocation)|rof(?:Loc|ile(?:Iterate(?:Data|ProcPtr|UPP)|Location|MD5(?:Ptr)?|Ref|SequenceDescType)))|RGBColor|S(?:15Fixed16ArrayType|creening(?:ChannelRec|Type)|ignatureType)|T(?:ag(?:ElemTable|Record)|ext(?:DescriptionType|Type))|U(?:16Fixed16ArrayType|Int(?:16ArrayType|32ArrayType|64ArrayType|8ArrayType)|crBgType|nicodeTextType)|Vi(?:deoCardGamma(?:Formula|T(?:able|ype))?|ewingConditionsType)|WorldRef|XYZType|YxyColor)|NCM(?:ConcatProfileS(?:et|pec)|DeviceProfileInfo))\\b",
+ "name": "invalid.deprecated.10.6.support.type.c"
+ },
+ {
+ "match": "\\bkC(?:FStream(?:PropertySSLPeerCertificates|SSLAllows(?:AnyRoot|Expired(?:Certificates|Roots)))|VImageBufferTransferFunction_(?:EBU_3213|SMPTE_C))\\b",
+ "name": "invalid.deprecated.10.6.support.variable.c"
+ },
+ {
+ "match": "\\b(?:AudioFileFDFTable(?:Extended)?|C(?:E_(?:A(?:ccessDescription|uthority(?:InfoAccess|KeyID))|BasicConstraints|C(?:RLDist(?:PointsSyntax|ributionPoint)|ertPolicies|rl(?:Dist(?:ReasonFlags|ributionPointNameType)|Reason))|D(?:ata(?:AndType)?|istributionPointName)|General(?:Name(?:s)?|Subtree(?:s)?)|I(?:nhibitAnyPolicy|ssuingDistributionPoint)|KeyUsage|N(?:ame(?:Constraints|RegistrationAuthorities)|etscapeCertType)|OtherName|Policy(?:Constraints|Information|Mapping(?:s)?|QualifierInfo)|QC_Statement(?:s)?|S(?:emanticsInformation|ubjectKeyID))|SSM_(?:A(?:C(?:CESS_CREDENTIALS(?:_PTR)?|L_(?:E(?:DIT(?:_PTR)?|NTRY_(?:IN(?:FO(?:_PTR)?|PUT(?:_PTR)?)|PROTOTYPE(?:_PTR)?))|OWNER_PROTOTYPE(?:_PTR)?|SUBJECT_CALLBACK|VALIDITY_PERIOD(?:_PTR)?))|PI_M(?:EMORY_FUNCS(?:_PTR)?|oduleEventHandler)|UTHORIZATIONGROUP(?:_PTR)?)|BASE_CERTS(?:_PTR)?|C(?:ALLBACK|ERT(?:GROUP(?:_PTR)?|_(?:BUNDLE(?:_(?:HEADER(?:_PTR)?|PTR))?|PAIR(?:_PTR)?))|HALLENGE_CALLBACK|ONTEXT(?:_(?:ATTRIBUTE(?:_PTR)?|PTR))?|R(?:L(?:GROUP(?:_PTR)?|_PAIR(?:_PTR)?)|YPTO_DATA(?:_PTR)?)|SP_OPERATIONAL_STATISTICS(?:_PTR)?)|D(?:AT(?:A_PTR|E(?:_PTR)?)|B(?:INFO(?:_PTR)?|_(?:ATTRIBUTE_(?:DATA(?:_PTR)?|INFO(?:_PTR)?)|INDEX_INFO(?:_PTR)?|PARSING_MODULE_INFO(?:_PTR)?|RECORD_(?:ATTRIBUTE_(?:DATA(?:_PTR)?|INFO(?:_PTR)?)|INDEX_INFO(?:_PTR)?)|SCHEMA_(?:ATTRIBUTE_INFO(?:_PTR)?|INDEX_INFO(?:_PTR)?)|UNIQUE_RECORD(?:_PTR)?))|L_DB_(?:HANDLE(?:_PTR)?|LIST(?:_PTR)?))|E(?:NCODED_C(?:ERT(?:_PTR)?|RL(?:_PTR)?)|VIDENCE(?:_PTR)?)|F(?:IELD(?:GROUP(?:_PTR)?|_PTR)?|UNC_NAME_ADDR(?:_PTR)?)|GUID(?:_PTR)?|K(?:E(?:A_DERIVE_PARAMS(?:_PTR)?|Y(?:HEADER(?:_PTR)?|_(?:PTR|SIZE(?:_PTR)?))?)|R(?:SUBSERVICE(?:_PTR)?|_(?:NAME|P(?:OLICY_(?:INFO(?:_PTR)?|LIST_ITEM(?:_PTR)?)|ROFILE(?:_PTR)?)|WRAPPEDPRODUCT_INFO(?:_PTR)?)))|LIST(?:_(?:ELEMENT|PTR))?|M(?:ANAGER_(?:EVENT_NOTIFICATION(?:_PTR)?|REGISTRATION_INFO(?:_PTR)?)|EMORY_FUNCS(?:_PTR)?|ODULE_FUNCS(?:_PTR)?)|N(?:AME_LIST(?:_PTR)?|ET_ADDRESS(?:_PTR)?)|OID_PTR|P(?:ARSED_C(?:ERT(?:_PTR)?|RL(?:_PTR)?)|KCS(?:1_OAEP_PARAMS(?:_PTR)?|5_PBKDF(?:1_PARAMS(?:_PTR)?|2_PARAMS(?:_PTR)?)))|QUERY(?:_(?:LIMITS(?:_PTR)?|PTR|SIZE_DATA(?:_PTR)?))?|R(?:ANGE(?:_PTR)?|ESOURCE_CONTROL_CONTEXT(?:_PTR)?)|S(?:AMPLE(?:GROUP(?:_PTR)?|_PTR)?|ELECTION_PREDICATE(?:_PTR)?|PI_(?:AC_FUNCS(?:_PTR)?|C(?:L_FUNCS(?:_PTR)?|SP_FUNCS(?:_PTR)?)|DL_FUNCS(?:_PTR)?|KR_FUNCS(?:_PTR)?|ModuleEventHandler|TP_FUNCS(?:_PTR)?)|TATE_FUNCS(?:_PTR)?|UBSERVICE_UID(?:_PTR)?)|T(?:P_(?:A(?:PPLE_EVIDENCE_INFO|UTHORITY_ID(?:_PTR)?)|C(?:ALLERAUTH_CONTEXT(?:_PTR)?|ERT(?:CHANGE_(?:INPUT(?:_PTR)?|OUTPUT(?:_PTR)?)|ISSUE_(?:INPUT(?:_PTR)?|OUTPUT(?:_PTR)?)|NOTARIZE_(?:INPUT(?:_PTR)?|OUTPUT(?:_PTR)?)|RECLAIM_(?:INPUT(?:_PTR)?|OUTPUT(?:_PTR)?)|VERIFY_(?:INPUT(?:_PTR)?|OUTPUT(?:_PTR)?))|ONFIRM_RESPONSE(?:_PTR)?|RLISSUE_(?:INPUT(?:_PTR)?|OUTPUT(?:_PTR)?))|POLICYINFO(?:_PTR)?|RE(?:QUEST_SET(?:_PTR)?|SULT_SET(?:_PTR)?)|VERIF(?:ICATION_RESULTS_CALLBACK|Y_CONTEXT(?:_(?:PTR|RESULT(?:_PTR)?))?))|UPLE(?:GROUP(?:_PTR)?|_PTR)?)|UPCALLS(?:_(?:CALLOC|FREE|MALLOC|PTR|REALLOC))?|VERSION(?:_PTR)?|WRAP_KEY(?:_PTR)?|X509(?:EXT_(?:BASICCONSTRAINTS(?:_PTR)?|P(?:AIR(?:_PTR)?|OLICY(?:INFO(?:_PTR)?|QUALIFIER(?:INFO(?:_PTR)?|S(?:_PTR)?)))|TAGandVALUE(?:_PTR)?)|_(?:ALGORITHM_IDENTIFIER_PTR|EXTENSION(?:S(?:_PTR)?|_PTR)?|NAME(?:_PTR)?|R(?:DN(?:_PTR)?|EVOKED_CERT_(?:ENTRY(?:_PTR)?|LIST(?:_PTR)?))|S(?:IGN(?:ATURE(?:_PTR)?|ED_C(?:ERTIFICATE(?:_PTR)?|RL(?:_PTR)?))|UBJECT_PUBLIC_KEY_INFO_PTR)|T(?:BS_CERT(?:IFICATE(?:_PTR)?|LIST(?:_PTR)?)|IME(?:_PTR)?|YPE_VALUE_PAIR(?:_PTR)?)|VALIDITY(?:_PTR)?))))|MDS_(?:DB_HANDLE|FUNCS(?:_PTR)?)|cssm_(?:ac(?:cess_credentials|l_(?:e(?:dit|ntry_(?:in(?:fo|put)|prototype))|owner_prototype|validity_period))|base_certs|c(?:ert(?:_(?:bundle(?:_header)?|pair)|group)|ontext(?:_attribute)?|r(?:l(?:_pair|group)|ypto_data))|d(?:b(?:_(?:attribute_(?:data|info)|index_info|parsing_module_info|record_(?:attribute_(?:data|info)|index_info)|schema_attribute_info|unique_record)|info)|l_db_list)|e(?:ncoded_c(?:ert|rl)|vidence)|field(?:group)?|k(?:e(?:a_derive_params|y(?:header)?)|r(?:_(?:p(?:olicy_(?:info|list_item)|rofile)|wrappedproductinfo)|subservice))|list_element|m(?:anager_(?:event_notification|registration_info)|odule_funcs)|net_address|pkcs(?:1_oaep_params|5_pbkdf(?:1_params|2_params))|query(?:_limits)?|resource_control_context|s(?:ample(?:group)?|election_predicate|pi_(?:ac_funcs|c(?:l_funcs|sp_funcs)|dl_funcs|kr_funcs|tp_funcs)|tate_funcs|ubservice_uid)|t(?:p_(?:authority_id|c(?:allerauth_context|ert(?:change_(?:input|output)|issue_(?:input|output)|notarize_(?:input|output)|reclaim_(?:input|output)|verify_(?:input|output))|onfirm_response|rlissue_(?:input|output))|policyinfo|request_set|verify_context(?:_result)?)|uplegroup)|upcalls|x509(?:_(?:extension(?:TagAndValue|s)?|name|r(?:dn|evoked_cert_(?:entry|list))|sign(?:ature|ed_c(?:ertificate|rl))|t(?:bs_cert(?:ificate|list)|ime|ype_value_pair))|ext_(?:basicConstraints|p(?:air|olicy(?:Info|Qualifier(?:Info|s))))))|mds_funcs|x509_validity)\\b",
+ "name": "invalid.deprecated.10.7.support.type.c"
+ },
+ {
+ "match": "\\b(?:CSSMOID_(?:A(?:D(?:C_CERT_POLICY|_(?:CA_(?:ISSUERS|REPOSITORY)|OCSP|TIME_STAMPING))|NSI_(?:DH_(?:EPHEM(?:_SHA1)?|HYBRID(?:1(?:_SHA1)?|2(?:_SHA1)?|_ONEFLOW)|ONE_FLOW(?:_SHA1)?|PUB_NUMBER|STATIC(?:_SHA1)?)|MQV(?:1(?:_SHA1)?|2(?:_SHA1)?))|PPLE(?:ID_(?:CERT_POLICY|SHARING_CERT_POLICY)|_(?:ASC|CERT_POLICY|E(?:CDSA|KU_(?:CODE_SIGNING(?:_DEV)?|ICHAT_(?:ENCRYPTION|SIGNING)|P(?:ASSBOOK_SIGNING|ROFILE_SIGNING)|QA_PROFILE_SIGNING|RESOURCE_SIGNING|SYSTEM_IDENTITY)|XTENSION(?:_(?:A(?:AI_INTERMEDIATE|DC_(?:APPLE_SIGNING|DEV_SIGNING)|PPLE(?:ID_(?:INTERMEDIATE|SHARING)|_SIGNING))|CODE_SIGNING|DEVELOPER_AUTHENTICATION|ESCROW_SERVICE|I(?:NTERMEDIATE_MARKER|TMS_INTERMEDIATE)|MACAPPSTORE_RECEIPT|P(?:ASSBOOK_SIGNING|ROVISIONING_PROFILE_SIGNING)|S(?:ERVER_AUTHENTICATION|YSINT2_INTERMEDIATE)|WWDR_INTERMEDIATE))?)|FEE(?:D(?:EXP)?|_(?:MD5|SHA1))?|ISIGN|TP_(?:APPLEID_SHARING|C(?:ODE_SIGN(?:ING)?|SR_GEN)|E(?:AP|SCROW_SERVICE)|I(?:CHAT|P_SEC)|LOCAL_CERT_GEN|M(?:ACAPPSTORE_RECEIPT|OBILE_STORE)|P(?:A(?:CKAGE_SIGNING|SSBOOK_SIGNING)|CS_ESCROW_SERVICE|KINIT_(?:CLIENT|SERVER)|RO(?:FILE_SIGNING|VISIONING_PROFILE_SIGNING))|QA_PROFILE_SIGNING|RE(?:SOURCE_SIGN|VOCATION(?:_(?:CRL|OCSP))?)|S(?:MIME|SL|W_UPDATE_SIGNING)|T(?:EST_MOBILE_STORE|IMESTAMPING))|X509_BASIC))|liasedEntryName|uthority(?:InfoAccess|KeyIdentifier|RevocationList))|B(?:asicConstraints|iometricInfo|usinessCategory)|C(?:ACertificate|SSMKeyStruct|ert(?:Issuer|i(?:com(?:EllCurve)?|ficate(?:Policies|RevocationList)))|hallengePassword|lientAuth|o(?:llective(?:FacsimileTelephoneNumber|InternationalISDNNumber|Organization(?:Name|alUnitName)|P(?:hysicalDeliveryOfficeName|ost(?:OfficeBox|al(?:Address|Code)))|St(?:ateProvinceName|reetAddress)|Tele(?:phoneNumber|x(?:Number|TerminalIdentifier)))|mmonName|ntentType|unt(?:erSignature|ryName))|r(?:l(?:DistributionPoints|Number|Reason)|ossCertificatePair))|D(?:ES_CBC|H|NQualifier|OTMAC_CERT(?:_(?:E(?:MAIL_(?:ENCRYPT|SIGN)|XTENSION)|IDENTITY|POLICY|REQ(?:_(?:ARCHIVE_(?:FETCH|LIST|REMOVE|STORE)|EMAIL_(?:ENCRYPT|SIGN)|IDENTITY|SHARED_SERVICES|VALUE_(?:ASYNC|HOSTNAME|IS_PENDING|PASSWORD|RENEW|USERNAME)))?))?|SA(?:_(?:CMS|JDK))?|e(?:ltaCrlIndicator|s(?:cription|tinationIndicator))|istinguishedName|omainComponent)|E(?:CDSA_WithS(?:HA(?:1|2(?:24|56)|384|512)|pecified)|KU_IPSec|TSI_QCS_QC_(?:COMPLIANCE|LIMIT_VALUE|RETENTION|SSCD)|mail(?:Address|Protection)|nhancedSearchGuide|xtended(?:CertificateAttributes|KeyUsage(?:Any)?|UseCodeSigning))|FacsimileTelephoneNumber|G(?:enerationQualifier|ivenName)|Ho(?:ldInstructionCode|useIdentifier)|I(?:n(?:hibitAnyPolicy|itials|ternationalISDNNumber|validityDate)|ssu(?:erAltName|ingDistributionPoint(?:s)?))|K(?:ERBv5_PKINIT_(?:AUTH_DATA|DH_KEY_DATA|KP_(?:CLIENT_AUTH|KDC)|RKEY_DATA)|eyUsage|nowledgeInformation)|LocalityName|M(?:ACAPPSTORE_(?:CERT_POLICY|RECEIPT_CERT_POLICY)|D(?:2(?:WithRSA)?|4(?:WithRSA)?|5(?:WithRSA)?)|OBILE_STORE_SIGNING_POLICY|e(?:mber|ssageDigest)|icrosoftSGC)|N(?:ame(?:Constraints)?|etscape(?:Cert(?:Sequence|Type)|SGC))|O(?:AEP_(?:ID_PSPECIFIED|MGF1)|CSPSigning|ID_QCS_SYNTAX_V(?:1|2)|bjectClass|rganization(?:Name|alUnitName)|wner)|P(?:DA_(?:COUNTRY_(?:CITIZEN|RESIDENCE)|DATE_OF_BIRTH|GENDER|PLACE_OF_BIRTH)|K(?:CS(?:12_(?:c(?:ertBag|rlBag)|keyBag|pbe(?:WithSHAAnd(?:128BitRC(?:2CBC|4)|2Key3DESCBC|3Key3DESCBC|40BitRC4)|withSHAAnd40BitRC2CBC)|s(?:afeContentsBag|ecretBag|hroudedKeyBag))|3|5_(?:D(?:ES_EDE3_CBC|IGEST_ALG)|ENCRYPT_ALG|HMAC_SHA1|PB(?:ES2|KDF2|MAC1)|RC(?:2_CBC|5_CBC)|pbeWith(?:MD(?:2And(?:DES|RC2)|5And(?:DES|RC2))|SHA1And(?:DES|RC2)))|7_(?:D(?:ata(?:WithAttributes)?|igestedData)|En(?:crypted(?:Data|PrivateKeyInfo)|velopedData)|Signed(?:AndEnvelopedData|Data))|9_(?:C(?:ertTypes|rlTypes)|FriendlyName|Id_Ct_TSTInfo|LocalKeyId|SdsiCertificate|TimeStampToken|X509C(?:ertificate|rl)))|IX_OCSP(?:_(?:ARCHIVE_CUTOFF|BASIC|CRL|NO(?:CHECK|NCE)|RESPONSE|SERVICE_LOCATOR))?)|hysicalDeliveryOfficeName|o(?:licy(?:Constraints|Mappings)|st(?:OfficeBox|al(?:Address|Code)))|r(?:e(?:ferredDeliveryMethod|sentationAddress)|ivateKeyUsagePeriod|otocolInformation))|Q(?:C_Statements|T_(?:CPS|UNOTICE))|R(?:SA(?:WithOAEP)?|egisteredAddress|oleOccupant)|S(?:HA(?:1(?:With(?:DSA(?:_(?:CMS|JDK))?|RSA(?:_OIW)?))?|2(?:24(?:WithRSA)?|56(?:WithRSA)?)|384(?:WithRSA)?|512(?:WithRSA)?)|e(?:archGuide|eAlso|r(?:ialNumber|verAuth))|igningTime|t(?:ateProvinceName|reetAddress)|u(?:bject(?:AltName|DirectoryAttributes|EmailAddress|InfoAccess|KeyIdentifier|Picture|SignatureBitmap)|pportedApplicationContext|rname))|T(?:EST_MOBILE_STORE_SIGNING_POLICY|ele(?:phoneNumber|x(?:Number|TerminalIdentifier))|i(?:meStamping|tle))|U(?:n(?:ique(?:Identifier|Member)|structured(?:Address|Name))|se(?:Exemptions|r(?:Certificate|ID|Password)))|X(?:509V(?:1(?:C(?:RL(?:Issuer(?:Name(?:CStruct|LDAP)|Struct)|N(?:extUpdate|umberOfRevokedCertEntries)|Revoked(?:Certificates(?:CStruct|Struct)|Entry(?:CStruct|RevocationDate|S(?:erialNumber|truct)))|ThisUpdate)|ertificate(?:IssuerUniqueId|SubjectUniqueId))|IssuerName(?:CStruct|LDAP|Std)?|S(?:erialNumber|ignature(?:Algorithm(?:Parameters|TBS)?|CStruct|Struct)?|ubject(?:Name(?:CStruct|LDAP|Std)?|PublicKey(?:Algorithm(?:Parameters)?|CStruct)?))|V(?:alidityNot(?:After|Before)|ersion))|2CRL(?:AllExtensions(?:CStruct|Struct)|Extension(?:Critical|Id|Type)|NumberOfExtensions|RevokedEntry(?:AllExtensions(?:CStruct|Struct)|Extension(?:Critical|Id|Type|Value)|NumberOfExtensions|SingleExtension(?:CStruct|Struct))|Si(?:gnedCrl(?:CStruct|Struct)|ngleExtension(?:CStruct|Struct))|TbsCertList(?:CStruct|Struct)|Version)|3(?:Certificate(?:CStruct|Extension(?:C(?:Struct|ritical)|Id|Struct|Type|Value|s(?:CStruct|Struct))|NumberOfExtensions)?|SignedCertificate(?:CStruct)?))|9_62(?:_(?:C_TwoCurve|EllCurve|FieldType|P(?:rimeCurve|ubKeyType)|SigType))?|_121Address)|ecPublicKey|sec(?:p(?:1(?:12r(?:1|2)|28r(?:1|2)|60(?:k1|r(?:1|2))|92(?:k1|r1))|2(?:24(?:k1|r1)|56(?:k1|r1))|384r1|521r1)|t(?:1(?:13r(?:1|2)|31r(?:1|2)|63(?:k1|r(?:1|2))|93r(?:1|2))|2(?:3(?:3(?:k1|r1)|9k1)|83(?:k1|r1))|409(?:k1|r1)|571(?:k1|r1))))|k(?:MDItemLabel(?:I(?:D|con)|Kind|UUID)|SCPropNetSMBNetBIOSScope))\\b",
+ "name": "invalid.deprecated.10.7.support.variable.c"
+ },
+ {
+ "match": "\\b(?:false32b|gestaltSystemVersion(?:BugFix|M(?:ajor|inor))?|kCT(?:FontTableOptionExcludeSynthetic|ParagraphStyleSpecifierLineSpacing)|true32b)\\b",
+ "name": "invalid.deprecated.10.8.support.constant.c"
+ },
+ {
+ "match": "\\bWSMethodInvocation(?:CallBackProcPtr|DeserializationProcPtr|SerializationProcPtr)\\b",
+ "name": "invalid.deprecated.10.8.support.type.c"
+ },
+ {
+ "match": "\\b(?:k(?:CTTypesetterOptionDisableBidiProcessing|FSOperation(?:Bytes(?:CompleteKey|RemainingKey)|Objects(?:CompleteKey|RemainingKey)|T(?:hroughputKey|otal(?:BytesKey|ObjectsKey|UserVisibleObjectsKey))|UserVisibleObjects(?:CompleteKey|RemainingKey))|LSSharedFileListVolumesIDiskVisible|WS(?:Debug(?:Incoming(?:Body|Headers)|Outgoing(?:Body|Headers))|Fault(?:Code|Extra|String)|HTTP(?:ExtraHeaders|FollowsRedirects|Message|Proxy|ResponseMessage|Version)|MethodInvocation(?:Result(?:ParameterName)?|TimeoutValue)|NetworkStreamFaultString|Record(?:NamespaceURI|ParameterOrder|Type)|S(?:OAP(?:1999Protocol|2001Protocol|BodyEncodingStyle|Me(?:ssageHeaders|thodNamespaceURI)|Style(?:Doc|RPC))|treamError(?:Domain|Error|Message))|XMLRPCProtocol))|pi)\\b",
+ "name": "invalid.deprecated.10.8.support.variable.c"
+ },
{
"match": "\\bkCFURLUbiquitousItemPercent(?:DownloadedKey|UploadedKey)\\b",
"name": "invalid.deprecated.10.8.support.variable.cf.c"
@@ -29,6 +137,10 @@
"match": "\\bkCGWindowWorkspace\\b",
"name": "invalid.deprecated.10.8.support.variable.quartz.c"
},
+ {
+ "match": "\\bk(?:AUVoiceIOProperty_VoiceProcessingQuality|Sec(?:AppleSharePasswordItemClass|TrustResultConfirm))\\b",
+ "name": "invalid.deprecated.10.9.support.constant.c"
+ },
{
"match": "\\bkCFURL(?:BookmarkCreationPreferFileIDResolutionMask|HFSPathStyle|ImproperArgumentsError|PropertyKeyUnavailableError|Re(?:moteHostUnavailableError|source(?:AccessViolationError|NotFoundError))|TimeoutError|Unknown(?:Error|PropertyKeyError|SchemeError))\\b",
"name": "invalid.deprecated.10.9.support.constant.cf.c"
@@ -38,17 +150,57 @@
"name": "invalid.deprecated.10.9.support.constant.quartz.c"
},
{
- "match": "\\bCFURLError\\b",
- "name": "invalid.deprecated.10.9.support.type.cf.c"
+ "match": "\\bSecTrustUserSetting\\b",
+ "name": "invalid.deprecated.10.9.support.type.c"
},
{
- "match": "\\bCGTextEncoding\\b",
- "name": "invalid.deprecated.10.9.support.type.quartz.c"
+ "match": "\\b(?:XPC_ACTIVITY_REQUIRE_(?:BATTERY_LEVEL|HDD_SPINNING)|k(?:LSSharedFileListGlobalLoginItems|S(?:C(?:PropNetAirPort(?:A(?:llowNetCreation|uthPassword(?:Encryption)?)|JoinMode|P(?:owerEnabled|referredNetwork)|SavePasswords)|ValNetAirPort(?:AuthPasswordEncryptionKeychain|JoinMode(?:Automatic|Preferred|R(?:anked|ecent)|Strongest)))|ecPolicyAppleiChat)))\\b",
+ "name": "invalid.deprecated.10.9.support.variable.c"
},
{
"match": "\\bkCFURL(?:File(?:DirectoryContents|Exists|L(?:astModificationTime|ength)|OwnerID|POSIXMode)|HTTPStatus(?:Code|Line)|UbiquitousItemIsDownloadedKey)\\b",
"name": "invalid.deprecated.10.9.support.variable.cf.c"
},
+ {
+ "match": "\\bk3DMixerParam_(?:GlobalReverbGain|M(?:axGain|inGain)|O(?:bstructionAttenuation|cclusionAttenuation)|ReverbBlend)\\b",
+ "name": "invalid.deprecated.tba.support.constant.c"
+ },
+ {
+ "match": "\\bkQL(?:Preview(?:ContentIDScheme|OptionCursorKey|Property(?:Attachment(?:DataKey|sKey)|BaseBundlePathKey|CursorKey|DisplayNameKey|HeightKey|MIMETypeKey|PDFStyleKey|StringEncodingKey|WidthKey))|ThumbnailProperty(?:Ba(?:dgeImageKey|seBundlePathKey)|ExtensionKey))\\b",
+ "name": "invalid.deprecated.tba.support.variable.c"
+ },
+ {
+ "match": "\\b(?:QOS_CLASS_(?:BACKGROUND|DEFAULT|U(?:NSPECIFIED|SER_IN(?:ITIATED|TERACTIVE)|TILITY))|k(?:C(?:GLCP(?:AbortOnGPURestartStatusBlacklisted|ContextPriorityRequest|GPURestartStatus|Support(?:GPURestart|SeparateAddressSpace))|TRuby(?:Alignment(?:Auto|Center|Distribute(?:Letter|Space)|End|Invalid|LineEdge|Start)|Overhang(?:Auto|End|Invalid|None|Start)|Position(?:After|Before|Count|In(?:line|terCharacter))))|FSEventStreamEventFlagItemIs(?:Hardlink|LastHardlink)|SecAccessControlUserPresence))\\b",
+ "name": "support.constant.10.10.c"
+ },
+ {
+ "match": "\\bk(?:A(?:XValueType(?:AXError|C(?:FRange|G(?:Point|Rect|Size))|Illegal)|udioComponent(?:Flag_(?:CanLoadInProcess|IsV3AudioUnit|RequiresAsyncInstantiation)|Instantiation_Load(?:InProcess|OutOfProcess)))|SecAccessControlDevicePasscode)\\b",
+ "name": "support.constant.10.11.c"
+ },
+ {
+ "match": "\\bkSec(?:AccessControl(?:A(?:nd|pplicationPassword)|Or|PrivateKeyUsage)|KeyOperationType(?:Decrypt|Encrypt|KeyExchange|Sign|Verify))\\b",
+ "name": "support.constant.10.12.c"
+ },
+ {
+ "match": "\\bk(?:CGLRPRe(?:gistryID(?:High|Low)|movable)|FSEventStream(?:CreateFlagUseExtendedData|EventFlagItemCloned)|SecAccessControlBiometry(?:Any|CurrentSet))\\b",
+ "name": "support.constant.10.13.c"
+ },
+ {
+ "match": "\\bk(?:3DMixerParam_(?:BusEnable|DryWetReverbBlend|GlobalReverbGainInDecibels|M(?:axGainInDecibels|inGainInDecibels)|O(?:bstructionAttenuationInDecibels|cclusionAttenuationInDecibels))|AudioQueueProperty_ChannelAssignments|JSTypeSymbol|SecAccessControlWatch)\\b",
+ "name": "support.constant.10.15.c"
+ },
+ {
+ "match": "\\bk(?:AudioComponentFlag_SandboxSafe|CGL(?:PFASupportsAutomaticGraphicsSwitching|Renderer(?:ATIRadeonX4000ID|IntelHD5000ID)))\\b",
+ "name": "support.constant.10.8.c"
+ },
+ {
+ "match": "\\bk(?:AXPriority(?:High|Low|Medium)|CGL(?:OGLPVersion_GL4_Core|R(?:PMajorGLVersion|endererGeForceID))|FSEventStream(?:CreateFlagMarkSelf|EventFlagOwnEvent))\\b",
+ "name": "support.constant.10.9.c"
+ },
+ {
+ "match": "\\b(?:A(?:APNot(?:CreatedErr|FoundErr)|C(?:E(?:2Type|8Type)|L_(?:A(?:DD_(?:FILE|SUBDIRECTORY)|PPEND_DATA)|CHANGE_OWNER|DELETE(?:_CHILD)?|E(?:NTRY_(?:DIRECTORY_INHERIT|FILE_INHERIT|INHERITED|LIMIT_INHERIT|ONLY_INHERIT)|X(?:ECUTE|TENDED_(?:ALLOW|DENY)))|F(?:IRST_ENTRY|LAG_(?:DEFER_INHERIT|NO_INHERIT))|L(?:AST_ENTRY|IST_DIRECTORY)|NEXT_ENTRY|READ_(?:ATTRIBUTES|DATA|EXTATTRIBUTES|SECURITY)|S(?:EARCH|YNCHRONIZE)|TYPE_(?:A(?:CCESS|FS)|CODA|DEFAULT|EXTENDED|N(?:TFS|WFS))|UNDEFINED_TAG|WRITE_(?:ATTRIBUTES|DATA|EXTATTRIBUTES|SECURITY)))|IF(?:C(?:ID|Version1)|FID)|SD(?:Bad(?:ForkErr|HeaderErr)|EntryNotFoundErr)|VAudioSessionError(?:Code(?:BadParam|Cannot(?:InterruptOthers|Start(?:Playing|Recording))|ExpiredSession|I(?:n(?:compatibleCategory|sufficientPriority)|sBusy)|M(?:ediaServicesFailed|issingEntitlement)|None|ResourceNotAvailable|S(?:essionNotActive|iriIsRecording)|Unspecified)|InsufficientPriority)|nnotationID|ppl(?:eShareMediaType|icationSpecificID)|u(?:dioRecordingID|thorID))|C(?:DEFNFnd|E_CDNT_(?:FullName|NameRelativeToCrlIssuer)|S(?:SM(?:ERR_(?:A(?:C_(?:DEVICE_(?:FAILED|RESET)|FUNCTION_(?:FAILED|NOT_IMPLEMENTED)|IN(?:SUFFICIENT_CLIENT_IDENTIFICATION|TERNAL_ERROR|VALID_(?:BASE_ACLS|C(?:L_HANDLE|ONTEXT_HANDLE)|D(?:ATA|B_(?:HANDLE|LIST(?:_POINTER)?)|L_HANDLE)|ENCODING|INPUT_POINTER|OUTPUT_POINTER|P(?:ASSTHROUGH_ID|OINTER)|REQUEST(?:OR|_DESCRIPTOR)|T(?:P_HANDLE|UPLE_CREDENTIALS)|VALIDITY_PERIOD)|_DARK_WAKE)|M(?:DS_ERROR|EMORY_ERROR)|NO_USER_INTERACTION|OS_ACCESS_DENIED|SE(?:LF_CHECK_FAILED|RVICE_NOT_AVAILABLE)|USER_CANCELED)|PPLE(?:DL_(?:DISK_FULL|FILE_TOO_BIG|IN(?:COMPATIBLE_(?:DATABASE_BLOB|KEY_BLOB)|VALID_(?:DATABASE_BLOB|KEY_BLOB|OPEN_PARAMETERS))|QUOTA_EXCEEDED)|TP_(?:BAD_CERT_FROM_ISSUER|C(?:A_PIN_MISMATCH|ERT_NOT_FOUND_FROM_ISSUER|ODE_SIGN_DEVELOPMENT|RL_(?:BAD_URI|EXPIRED|INVALID_ANCHOR_CERT|NOT_(?:FOUND|TRUSTED|VALID_YET)|POLICY_FAIL|SERVER_DOWN)|S_(?:BAD_(?:CERT_CHAIN_LENGTH|PATH_LENGTH)|NO_(?:BASIC_CONSTRAINTS|EXTENDED_KEY_USAGE)))|EXT_KEYUSAGE_NOT_CRITICAL|HOSTNAME_MISMATCH|I(?:D(?:ENTIFIER_MISSING|P_FAIL)|N(?:COMPLETE_REVOCATION_CHECK|VALID_(?:AUTHORITY_ID|CA|E(?:MPTY_SUBJECT|XTENDED_KEY_USAGE)|ID_LINKAGE|KEY_USAGE|ROOT|SUBJECT_ID)))|MISSING_REQUIRED_EXTENSION|N(?:ETWORK_FAILURE|O_BASIC_CONSTRAINTS)|OCSP_(?:BAD_RE(?:QUEST|SPONSE)|INVALID_ANCHOR_CERT|NO(?:NCE_MISMATCH|T_TRUSTED|_SIGNER)|RESP_(?:INTERNAL_ERR|MALFORMED_REQ|SIG_REQUIRED|TRY_LATER|UNAUTHORIZED)|S(?:IG_ERROR|TATUS_UNRECOGNIZED)|UNAVAILABLE)|PATH_LEN_CONSTRAINT|RS_BAD_(?:CERT_CHAIN_LENGTH|EXTENDED_KEY_USAGE)|S(?:MIME_(?:BAD_(?:EXT_KEY_USE|KEY_USE)|EMAIL_ADDRS_NOT_FOUND|KEYUSAGE_NOT_CRITICAL|NO_EMAIL_ADDRS|SUBJ_ALT_NAME_NOT_CRIT)|SL_BAD_EXT_KEY_USE)|TRUST_SETTING_DENY|UNKNOWN_(?:C(?:ERT_EXTEN|R(?:ITICAL_EXTEN|L_EXTEN))|QUAL_CERT_STATEMENT))|_DOTMAC_(?:CSR_VERIFY_FAIL|FAILED_CONSISTENCY_CHECK|NO_REQ_PENDING|REQ_(?:IS_PENDING|QUEUED|REDIRECT|SERVER_(?:A(?:LREADY_EXIST|UTH)|ERR|NOT_AVAIL|PARAM|SERVICE_ERROR|UNIMPL)))))|C(?:L_(?:CRL_ALREADY_SIGNED|DEVICE_(?:FAILED|RESET)|FUNCTION_(?:FAILED|NOT_IMPLEMENTED)|IN(?:SUFFICIENT_CLIENT_IDENTIFICATION|TERNAL_ERROR|VALID_(?:BUNDLE_(?:INFO|POINTER)|C(?:ACHE_HANDLE|ERT(?:GROUP_POINTER|_POINTER)|ONTEXT_HANDLE|RL_(?:INDEX|POINTER))|DATA|FIELD_POINTER|INPUT_POINTER|NUMBER_OF_FIELDS|OUTPUT_POINTER|P(?:ASSTHROUGH_ID|OINTER)|RESULTS_HANDLE|SCOPE)|_DARK_WAKE)|M(?:DS_ERROR|EMORY_ERROR)|NO_(?:FIELD_VALUES|USER_INTERACTION)|OS_ACCESS_DENIED|S(?:COPE_NOT_SUPPORTED|E(?:LF_CHECK_FAILED|RVICE_NOT_AVAILABLE))|U(?:NKNOWN_(?:FORMAT|TAG)|SER_CANCELED)|VERIFICATION_FAILURE)|S(?:P(?:DL_APPLE_DL_CONVERSION_ERROR|_(?:A(?:CL_(?:ADD_FAILED|BASE_CERTS_NOT_SUPPORTED|CHA(?:LLENGE_CALLBACK_FAILED|NGE_FAILED)|DELETE_FAILED|ENTRY_TAG_NOT_FOUND|REPLACE_FAILED|SUBJECT_TYPE_NOT_SUPPORTED)|L(?:GID_MISMATCH|READY_LOGGED_IN)|PPLE_(?:ADD_APPLICATION_ACL_SUBJECT|INVALID_KEY_(?:END_DATE|START_DATE)|PUBLIC_KEY_INCOMPLETE|S(?:IGNATURE_MISMATCH|SLv2_ROLLBACK))|TTACH_HANDLE_BUSY)|BLOCK_SIZE_MISMATCH|CRYPTO_DATA_CALLBACK_FAILED|DEVICE_(?:ERROR|FAILED|MEMORY_ERROR|RESET|VERIFY_FAILED)|FUNCTION_(?:FAILED|NOT_IMPLEMENTED)|IN(?:PUT_LENGTH_ERROR|SUFFICIENT_CLIENT_IDENTIFICATION|TERNAL_ERROR|VALID_(?:A(?:C(?:CESS_CREDENTIALS|L_(?:BASE_CERTS|CHALLENGE_CALLBACK|E(?:DIT_MODE|NTRY_TAG)|SUBJECT_VALUE))|LGORITHM|TTR_(?:A(?:CCESS_CREDENTIALS|LG_PARAMS)|B(?:ASE|LOCK_SIZE)|DL_DB_HANDLE|E(?:FFECTIVE_BITS|ND_DATE)|I(?:NIT_VECTOR|TERATION_COUNT)|KEY(?:_(?:LENGTH|TYPE))?|LABEL|MODE|OUTPUT_SIZE|P(?:A(?:DDING|SSPHRASE)|RI(?:ME|VATE_KEY_FORMAT)|UBLIC_KEY_FORMAT)|R(?:ANDOM|OUNDS)|S(?:ALT|EED|TART_DATE|UBPRIME|YMMETRIC_KEY_FORMAT)|VERSION|WRAPPED_KEY_FORMAT))|C(?:ONTEXT(?:_HANDLE)?|RYPTO_DATA)|D(?:ATA(?:_COUNT)?|IGEST_ALGORITHM)|INPUT_(?:POINTER|VECTOR)|KEY(?:ATTR_MASK|USAGE_MASK|_(?:CLASS|FORMAT|LABEL|POINTER|REFERENCE))?|LOGIN_NAME|NEW_ACL_(?:ENTRY|OWNER)|OUTPUT_(?:POINTER|VECTOR)|P(?:ASSTHROUGH_ID|OINTER)|S(?:AMPLE_VALUE|IGNATURE))|_DARK_WAKE)|KEY_(?:BLOB_TYPE_INCORRECT|HEADER_INCONSISTENT|LABEL_ALREADY_EXISTS|USAGE_INCORRECT)|M(?:DS_ERROR|EMORY_ERROR|ISSING_ATTR_(?:A(?:CCESS_CREDENTIALS|LG_PARAMS)|B(?:ASE|LOCK_SIZE)|DL_DB_HANDLE|E(?:FFECTIVE_BITS|ND_DATE)|I(?:NIT_VECTOR|TERATION_COUNT)|KEY(?:_(?:LENGTH|TYPE))?|LABEL|MODE|OUTPUT_SIZE|P(?:A(?:DDING|SSPHRASE)|RI(?:ME|VATE_KEY_FORMAT)|UBLIC_KEY_FORMAT)|R(?:ANDOM|OUNDS)|S(?:ALT|EED|TART_DATE|UBPRIME|YMMETRIC_KEY_FORMAT)|VERSION|WRAPPED_KEY_FORMAT))|NO(?:T_LOGGED_IN|_USER_INTERACTION)|O(?:BJECT_(?:ACL_(?:NOT_SUPPORTED|REQUIRED)|MANIP_AUTH_DENIED|USE_AUTH_DENIED)|PERATION_AUTH_DENIED|S_ACCESS_DENIED|UTPUT_LENGTH_ERROR)|P(?:RIV(?:ATE_KEY_(?:ALREADY_EXISTS|NOT_FOUND)|ILEGE_NOT_(?:GRANTED|SUPPORTED))|UBLIC_KEY_INCONSISTENT)|QUERY_SIZE_UNKNOWN|S(?:AMPLE_VALUE_NOT_SUPPORTED|E(?:LF_CHECK_FAILED|RVICE_NOT_AVAILABLE)|TAGED_OPERATION_(?:IN_PROGRESS|NOT_STARTED))|U(?:NSUPPORTED_KEY(?:ATTR_MASK|USAGE_MASK|_(?:FORMAT|LABEL|SIZE))|SER_CANCELED)|VE(?:CTOR_OF_BUFS_UNSUPPORTED|RIFY_FAILED)))|SM_(?:A(?:DDIN_(?:AUTHENTICATE_FAILED|LOAD_FAILED|UNLOAD_FAILED)|TTRIBUTE_NOT_IN_CONTEXT)|BUFFER_TOO_SMALL|DEVICE_(?:FAILED|RESET)|E(?:MM_(?:AUTHENTICATE_FAILED|LOAD_FAILED|UNLOAD_FAILED)|VENT_NOTIFICATION_CALLBACK_NOT_FOUND)|FUNCTION_(?:FAILED|INTEGRITY_FAIL|NOT_IMPLEMENTED)|IN(?:COMPATIBLE_VERSION|SUFFICIENT_CLIENT_IDENTIFICATION|TERNAL_ERROR|VALID_(?:A(?:DDIN_(?:FUNCTION_TABLE|HANDLE)|TTRIBUTE)|CONTEXT_HANDLE|GUID|HANDLE_USAGE|INPUT_POINTER|KEY_HIERARCHY|OUTPUT_POINTER|P(?:OINTER|VC)|S(?:ERVICE_MASK|UBSERVICEID))|_DARK_WAKE)|LIB_REF_NOT_FOUND|M(?:DS_ERROR|EMORY_ERROR|ODULE_(?:MAN(?:AGER_(?:INITIALIZE_FAIL|NOT_FOUND)|IFEST_VERIFY_FAILED)|NOT_LOADED))|NO(?:T_INITIALIZED|_USER_INTERACTION)|OS_ACCESS_DENIED|P(?:RIVILEGE_NOT_GRANTED|VC_(?:ALREADY_CONFIGURED|REFERENT_NOT_FOUND))|S(?:COPE_NOT_SUPPORTED|E(?:LF_CHECK_FAILED|RVICE_NOT_AVAILABLE))|USER_CANCELED)))|DL_(?:ACL_(?:ADD_FAILED|BASE_CERTS_NOT_SUPPORTED|CHA(?:LLENGE_CALLBACK_FAILED|NGE_FAILED)|DELETE_FAILED|ENTRY_TAG_NOT_FOUND|REPLACE_FAILED|SUBJECT_TYPE_NOT_SUPPORTED)|D(?:ATA(?:BASE_CORRUPT|STORE_(?:ALREADY_EXISTS|DOESNOT_EXIST|IS_OPEN))|B_LOCKED|EVICE_(?:FAILED|RESET))|ENDOFDATA|F(?:IELD_SPECIFIED_MULTIPLE|UNCTION_(?:FAILED|NOT_IMPLEMENTED))|IN(?:COMPATIBLE_FIELD_FORMAT|SUFFICIENT_CLIENT_IDENTIFICATION|TERNAL_ERROR|VALID_(?:AC(?:CESS_(?:CREDENTIALS|REQUEST)|L_(?:BASE_CERTS|CHALLENGE_CALLBACK|E(?:DIT_MODE|NTRY_TAG)|SUBJECT_VALUE))|C(?:L_HANDLE|SP_HANDLE)|D(?:B_(?:HANDLE|L(?:IST_POINTER|OCATION)|NAME)|L_HANDLE)|FIELD_NAME|IN(?:DEX_INFO|PUT_POINTER)|MODIFY_MODE|NE(?:TWORK_ADDR|W_(?:ACL_(?:ENTRY|OWNER)|OWNER))|O(?:PEN_PARAMETERS|UTPUT_POINTER)|P(?:A(?:RSING_MODULE|SSTHROUGH_ID)|OINTER)|QUERY|RE(?:CORD(?:TYPE|_(?:INDEX|UID))|SULTS_HANDLE)|S(?:AMPLE_VALUE|ELECTION_TAG)|UNIQUE_INDEX_DATA|VALUE)|_DARK_WAKE)|M(?:DS_ERROR|EMORY_ERROR|ISSING_VALUE|ULTIPLE_VALUES_UNSUPPORTED)|NO_USER_INTERACTION|O(?:BJECT_(?:ACL_(?:NOT_SUPPORTED|REQUIRED)|MANIP_AUTH_DENIED|USE_AUTH_DENIED)|PERATION_AUTH_DENIED|S_ACCESS_DENIED)|RECORD_(?:MODIFIED|NOT_FOUND)|S(?:AMPLE_VALUE_NOT_SUPPORTED|E(?:LF_CHECK_FAILED|RVICE_NOT_AVAILABLE)|TALE_UNIQUE_RECORD)|U(?:NSUPPORTED_(?:FIELD_FORMAT|INDEX_INFO|LOCALITY|NUM_(?:ATTRIBUTES|INDEXES|RECORDTYPES|SELECTION_PREDS)|OPERATOR|QUERY(?:_LIMITS)?|RECORDTYPE)|SER_CANCELED))|TP_(?:AUTHENTICATION_FAILED|C(?:ERT(?:GROUP_INCOMPLETE|IFICATE_CANT_OPERATE|_(?:EXPIRED|NOT_VALID_YET|REVOKED|SUSPENDED))|RL_ALREADY_SIGNED)|DEVICE_(?:FAILED|RESET)|FUNCTION_(?:FAILED|NOT_IMPLEMENTED)|IN(?:SUFFICIENT_C(?:LIENT_IDENTIFICATION|REDENTIALS)|TERNAL_ERROR|VALID_(?:A(?:CTION(?:_DATA)?|NCHOR_CERT|UTHORITY)|C(?:ALL(?:BACK|ERAUTH_CONTEXT_POINTER)|ERT(?:GROUP(?:_POINTER)?|IFICATE|_(?:AUTHORITY|POINTER))|L_HANDLE|ONTEXT_HANDLE|RL(?:GROUP(?:_POINTER)?|_(?:AUTHORITY|ENCODING|POINTER|TYPE))?|SP_HANDLE)|D(?:ATA|B_(?:HANDLE|LIST(?:_POINTER)?)|L_HANDLE)|F(?:IELD_POINTER|ORM_TYPE)|I(?:D(?:ENTIFIER(?:_POINTER)?)?|N(?:DEX|PUT_POINTER))|KEYCACHE_HANDLE|N(?:AME|ETWORK_ADDR|UMBER_OF_FIELDS)|OUTPUT_POINTER|P(?:ASSTHROUGH_ID|O(?:INTER|LICY_IDENTIFIERS))|RE(?:ASON|QUEST_INPUTS|SPONSE_VECTOR)|S(?:IGNATURE|TOP_ON_POLICY)|T(?:IMESTRING|UPLE(?:GROUP(?:_POINTER)?)?))|_DARK_WAKE)|M(?:DS_ERROR|EMORY_ERROR)|NO(?:T_(?:SIGNER|TRUSTED)|_(?:DEFAULT_AUTHORITY|USER_INTERACTION))|OS_ACCESS_DENIED|RE(?:JECTED_FORM|QUEST_(?:LOST|REJECTED))|SE(?:LF_CHECK_FAILED|RVICE_NOT_AVAILABLE)|U(?:N(?:KNOWN_(?:FORMAT|TAG)|SUPPORTED_(?:ADDR_TYPE|SERVICE))|SER_CANCELED)|VERIF(?:ICATION_FAILURE|Y_ACTION_FAILED)))|_(?:A(?:C(?:L_(?:AUTHORIZATION_(?:ANY|CHANGE_(?:ACL|OWNER)|D(?:B(?:S_(?:CREATE|DELETE)|_(?:DELETE|INSERT|MODIFY|READ))|E(?:CRYPT|LETE|RIVE))|E(?:NCRYPT|XPORT_(?:CLEAR|WRAPPED))|GENKEY|I(?:MPORT_(?:CLEAR|WRAPPED)|NTEGRITY)|LOGIN|MAC|P(?:ARTITION_ID|REAUTH_(?:BASE|END))|SIGN|TAG_VENDOR_DEFINED_START)|CODE_SIGNATURE_(?:INVALID|OSX)|EDIT_MODE_(?:ADD|DELETE|REPLACE)|KEYCHAIN_PROMPT_(?:CURRENT_VERSION|INVALID(?:_ACT)?|REQUIRE_PASSPHRASE|UNSIGNED(?:_ACT)?)|MATCH_(?:BITS|GID|HONOR_ROOT|UID)|PR(?:EAUTH_TRACKING_(?:AUTHORIZED|BLOCKED|COUNT_MASK|UNKNOWN)|OCESS_SELECTOR_CURRENT_VERSION)|SUBJECT_TYPE_(?:A(?:NY|SYMMETRIC_KEY)|BIOMETRIC|CO(?:DE_SIGNATURE|MMENT)|EXT_PAM_NAME|HASHED_SUBJECT|KEYCHAIN_PROMPT|LOGIN_NAME|P(?:A(?:RTITION|SSWORD)|R(?:EAUTH(?:_SOURCE)?|O(?:CESS|MPTED_(?:BIOMETRIC|PASSWORD)|TECTED_(?:BIOMETRIC|PASSWORD)))|UBLIC_KEY)|SYMMETRIC_KEY|THRESHOLD))|_(?:BASE_(?:AC_ERROR|ERROR)|PRIVATE_ERROR))|DDR_(?:CUSTOM|N(?:AME|ONE)|SOCKADDR|URL)|LG(?:CLASS_(?:ASYMMETRIC|CUSTOM|D(?:ERIVEKEY|IGEST)|KEYGEN|MAC|NONE|RANDOMGEN|S(?:IGNATURE|YMMETRIC)|UNIQUEGEN)|ID_(?:3DES(?:_(?:1KEY(?:_EEE)?|2KEY(?:_E(?:DE|EE))?|3KEY(?:_E(?:DE|EE))?))?|A(?:ES|PPLE_YARROW|SC)|B(?:ATON|LOWFISH)|C(?:AST(?:3|5)?|DMF|RAB|USTOM|oncat(?:BaseAnd(?:Data|Key)|DataAndBase|KeyAndBase))|D(?:ES(?:Random|X)?|H|SA(?:_BSAFE)?)|E(?:C(?:AES|C|D(?:H(?:_X963_KDF)?|SA(?:_SPECIFIED)?)|ES|MQV|NRA)|NTROPY_DEFAULT|lGamal|xtractFromKey)|F(?:ASTHASH|E(?:AL|E(?:D(?:EXP)?|_(?:MD5|SHA1))?)|IPS186Random|ortezzaTimestamp)|G(?:OST|enericSecret)|HAVAL(?:3|4|5)?|I(?:BCHASH|DEA|ntelPlatformRandom)|JUNIPER|K(?:E(?:A|YCHAIN_KEY)|H(?:AFRE|UFU))|L(?:AST|OKI|UCIFER)|M(?:A(?:DRYGA|YFLY)|D(?:2(?:Random|WithRSA)?|4|5(?:HMAC|Random|WithRSA)?)|MB|QV)|N(?:HASH|ONE|RA)|OPENSSH1|P(?:BE_OPENSSL_MD5|H|KCS(?:12_(?:PBE_(?:ENCR|MAC)|SHA1_PBE)|5_PBKDF(?:1_(?:MD(?:2|5)|SHA1)|2)))|R(?:C(?:2|4|5)|DES|EDOC(?:3)?|IPEM(?:AC|D)|SA|UNNING_COUNTER)|S(?:AFER|E(?:AL|CURE_PASSPHRASE)|HA(?:1(?:HMAC(?:_LEGACY)?|With(?:DSA|EC(?:DSA|NRA)|RSA))?|2(?:24(?:With(?:ECDSA|RSA))?|56(?:With(?:ECDSA|RSA))?)|384(?:With(?:ECDSA|RSA))?|512(?:With(?:ECDSA|RSA))?|Random)|KIPJACK|SL3(?:KeyAndMacDerive|M(?:D5(?:_MAC)?|asterDerive)|PreMasterGen|SHA1(?:_MAC)?))|TIGER|UTC|VENDOR_DEFINED|Wrap(?:Lynks|SET_OAEP)|XORBaseAndData|_FIRST_UNUSED)|MODE_(?:BC|C(?:BC(?:128|64|C|P(?:D|adIV8)|_IV8)?|FB(?:16|32|8|PadIV8|_IV8)?|OUNTER|USTOM)|ECB(?:128|64|96|Pad)?|ISO_9796|LAST|NONE|O(?:AEP_HASH|FB(?:64|NLF|PadIV8|_IV8)?)|P(?:BC|CBC|FB|KCS1_EM(?:E_(?:OAEP|V15)|SA_V15)|RIVATE_(?:KEY|WRAP)|UBLIC_KEY)|RELAYX|SHUFFLE|VENDOR_DEFINED|WRAP|X9_31))|PPLE(?:CSP(?:DL_DB_(?:CHANGE_PASSWORD|GET_(?:HANDLE|SETTINGS)|IS_LOCKED|LOCK|SET_SETTINGS|UNLOCK)|_KEYDIGEST)|DL_OPEN_PARAMETERS_VERSION|FILEDL_(?:COMMIT|DELETE_FILE|MAKE_(?:BACKUP|COPY)|ROLLBACK|T(?:AKE_FILE_LOCK|OGGLE_AUTOCOMMIT))|SCPDL_CSP_GET_KEYHANDLE|X509CL_(?:OBTAIN_CSR|VERIFY_CSR)|_(?:PRIVATE_CSPDL_CODE_(?:1(?:0|1|2|3|4|5|6|7|8|9)|2(?:0|1|2|3|4|5|6|7)|8|9)|UNLOCK_TYPE_(?:KEY(?:BAG|_DIRECT)|WRAPPED_PRIVATE)))|SC_OPTIMIZE_(?:ASCII|DEFAULT|S(?:ECURITY|IZE)|TIME(?:_SIZE)?)|TT(?:ACH_READ_ONLY|RIBUTE_(?:A(?:CCESS_CREDENTIALS|L(?:ERT_TITLE|G_(?:ID|PARAMS))|SC_OPTIMIZATION)|B(?:ASE|LOCK_SIZE)|C(?:SP_HANDLE|USTOM)|D(?:ATA_(?:ACCESS_CREDENTIALS|C(?:RYPTO_DATA|SSM_DATA)|D(?:ATE|L_DB_HANDLE)|K(?:EY|R_PROFILE)|NONE|RANGE|STRING|UINT32|VERSION)|ESCRIPTION|L_DB_HANDLE)|E(?:FFECTIVE_BITS|ND_DATE)|FEE_(?:CURVE_TYPE|PRIME_TYPE)|I(?:NIT_VECTOR|TERATION_COUNT|V_SIZE)|K(?:EY(?:ATTR|USAGE|_(?:LENGTH(?:_RANGE)?|TYPE))?|RPROFILE_(?:LOCAL|REMOTE))|LABEL|MODE|NONE|OUTPUT_SIZE|P(?:A(?:DDING|RAM_KEY|SSPHRASE)|R(?:I(?:ME|VATE_KEY_FORMAT)|OMPT)|UBLIC_KEY(?:_FORMAT)?)|R(?:ANDOM|OUNDS(?:_RANGE)?|SA_BLINDING)|S(?:ALT|EED|TART_DATE|UBPRIME|YMMETRIC_KEY_FORMAT)|TYPE_MASK|VE(?:NDOR_DEFINED|R(?:IFY_PASSPHRASE|SION))|WRAPPED_KEY_FORMAT)))|BASE_ERROR|C(?:ERT(?:GROUP_(?:CERT_PAIR|DATA|ENCODED_CERT|PARSED_CERT)|_(?:ACL_ENTRY|BUNDLE_(?:CUSTOM|ENCODING_(?:BER|CUSTOM|DER|PGP|SEXPR|UNKNOWN)|LAST|P(?:FX|GP_KEYRING|KCS(?:12|7_SIGNED_(?:DATA|ENVELOPED_DATA)))|SPKI_SEQUENCE|UNKNOWN)|ENCODING_(?:BER|CUSTOM|DER|LAST|MULTIPLE|NDR|PGP|SEXPR|UNKNOWN)|Intel|LAST|MULTIPLE|P(?:ARSE_FORMAT_(?:C(?:OMPLEX|USTOM)|LAST|MULTIPLE|NONE|OID_NAMED|SEXPR|TUPLE)|GP)|S(?:DSIv1|PKI|TATUS_(?:EXPIRED|IS_(?:FROM_NET|IN_(?:ANCHORS|INPUT_CERTS)|ROOT)|NOT_VALID_YET|TRUST_SETTINGS_(?:DENY|FOUND_(?:ADMIN|SYSTEM|USER)|IGNORED_ERROR|TRUST)))|TUPLE|UNKNOWN|X(?:9_ATTRIBUTE|_509(?:_ATTRIBUTE|v(?:1|2|3)))))|L_(?:BASE_(?:CL_ERROR|ERROR)|CUSTOM_C(?:ERT_(?:BUNDLE_TYPE|ENCODING|PARSE_FORMAT|TYPE)|RL_PARSE_FORMAT)|PRIVATE_ERROR|TEMPLATE_(?:INTERMEDIATE_CERT|PKIX_CERTTEMPLATE))|ONTEXT_EVENT_(?:CREATE|DELETE|UPDATE)|RL(?:GROUP_(?:CRL_PAIR|DATA|ENCODED_CRL|PARSED_CRL)|_(?:ENCODING_(?:B(?:ER|LOOM)|CUSTOM|DER|MULTIPLE|SEXPR|UNKNOWN)|PARSE_FORMAT_(?:C(?:OMPLEX|USTOM)|LAST|MULTIPLE|NONE|OID_NAMED|SEXPR|TUPLE)|TYPE_(?:MULTIPLE|SPKI|UNKNOWN|X_509v(?:1|2))))|S(?:P_(?:BASE_(?:CSP_ERROR|ERROR)|H(?:ARDWARE|YBRID)|PRIVATE_ERROR|RDR_(?:EXISTS|HW|TOKENPRESENT)|S(?:OFTWARE|TORES_(?:CERTIFICATES|GENERIC|P(?:RIVATE_KEYS|UBLIC_KEYS)|SESSION_KEYS))|TOK_(?:CLOCK_EXISTS|LOGIN_REQUIRED|PR(?:IVATE_KEY_PASSWORD|OT_AUTHENTICATION)|RNG|SESSION_KEY_PASSWORD|USER_PIN_(?:EXPIRED|INITIALIZED)|WRITE_PROTECTED))|SM_(?:BASE_(?:CSSM_ERROR|ERROR)|PRIVATE_ERROR))|USTOM_COMMON_ERROR_EXTENT)|D(?:B_(?:A(?:CCESS_(?:PRIVILEGED|RE(?:AD|SET)|WRITE)|ND|TTRIBUTE_(?:FORMAT_(?:B(?:IG_NUM|LOB)|COMPLEX|MULTI_UINT32|REAL|S(?:INT32|TRING)|TIME_DATE|UINT32)|NAME_AS_(?:INTEGER|OID|STRING)))|C(?:ERT_USE_(?:OWNER|PRIVACY|REVOKED|S(?:IGNING|YSTEM)|TRUSTED)|ONTAINS(?:_(?:FINAL_SUBSTRING|INITIAL_SUBSTRING))?)|DATASTORES_UNKNOWN|EQUAL|FILESYSTEMSCAN_MODE|GREATER_THAN|INDEX_(?:NONUNIQUE|ON_(?:ATTRIBUTE|RECORD|UNKNOWN)|UNIQUE)|LESS_THAN|MODIFY_ATTRIBUTE_(?:ADD|DELETE|NONE|REPLACE)|NO(?:NE|T_EQUAL)|OR|RECORDTYPE_(?:APP_DEFINED_(?:END|START)|OPEN_GROUP_(?:END|START)|SCHEMA_(?:END|START))|TRANSACTIONAL_MODE)|L_(?:BASE_(?:DL_ERROR|ERROR)|CUSTOM|DB_(?:RECORD_(?:A(?:LL_KEYS|NY|PPLESHARE_PASSWORD)|C(?:ERT|RL)|EXTENDED_ATTRIBUTE|GENERIC(?:_PASSWORD)?|INTERNET_PASSWORD|METADATA|P(?:OLICY|RIVATE_KEY|UBLIC_KEY)|SYMMETRIC_KEY|U(?:NLOCK_REFERRAL|SER_TRUST)|X509_C(?:ERTIFICATE|RL))|SCHEMA_(?:ATTRIBUTES|IN(?:DEXES|FO)|PARSING_MODULE))|FFS|LDAP|MEMORY|ODBC|P(?:KCS11|RIVATE_ERROR)|REMOTEDIR|UNKNOWN))|E(?:LAPSED_TIME_(?:COMPLETE|UNKNOWN)|RR(?:CODE_(?:ACL_(?:ADD_FAILED|BASE_CERTS_NOT_SUPPORTED|CHA(?:LLENGE_CALLBACK_FAILED|NGE_FAILED)|DELETE_FAILED|ENTRY_TAG_NOT_FOUND|REPLACE_FAILED|SUBJECT_TYPE_NOT_SUPPORTED)|CRL_ALREADY_SIGNED|DEVICE_(?:FAILED|RESET)|FUNCTION_(?:FAILED|NOT_IMPLEMENTED)|IN(?:COMPATIBLE_VERSION|SUFFICIENT_CLIENT_IDENTIFICATION|TERNAL_ERROR|VALID_(?:AC(?:CESS_CREDENTIALS|L_(?:BASE_CERTS|CHALLENGE_CALLBACK|E(?:DIT_MODE|NTRY_TAG)|SUBJECT_VALUE)|_HANDLE)|C(?:ERT(?:GROUP_POINTER|_POINTER)|L_HANDLE|ONTEXT_HANDLE|R(?:L_POINTER|YPTO_DATA)|SP_HANDLE)|D(?:ATA|B_(?:HANDLE|LIST(?:_POINTER)?)|L_HANDLE)|FIELD_POINTER|GUID|INPUT_POINTER|KR_HANDLE|N(?:E(?:TWORK_ADDR|W_ACL_(?:ENTRY|OWNER))|UMBER_OF_FIELDS)|OUTPUT_POINTER|P(?:ASSTHROUGH_ID|OINTER)|SAMPLE_VALUE|TP_HANDLE)|_DARK_WAKE)|M(?:DS_ERROR|EMORY_ERROR|ODULE_MANIFEST_VERIFY_FAILED)|NO_USER_INTERACTION|O(?:BJECT_(?:ACL_(?:NOT_SUPPORTED|REQUIRED)|MANIP_AUTH_DENIED|USE_AUTH_DENIED)|PERATION_AUTH_DENIED|S_ACCESS_DENIED)|PRIVILEGE_NOT_GRANTED|S(?:AMPLE_VALUE_NOT_SUPPORTED|E(?:LF_CHECK_FAILED|RVICE_NOT_AVAILABLE))|U(?:NKNOWN_(?:FORMAT|TAG)|SER_CANCELED)|VERIFICATION_FAILURE)|ORCODE_(?:C(?:OMMON_EXTENT|USTOM_OFFSET)|MODULE_EXTENT))|STIMATED_TIME_UNKNOWN|VIDENCE_FORM_(?:APPLE_(?:CERT(?:GROUP|_INFO)|HEADER)|C(?:ERT(?:_ID)?|RL(?:_(?:ID|NEXTTIME|THISTIME))?)|POLICYINFO|TUPLEGROUP|UNSPECIFIC|VERIFIER_TIME))|F(?:ALSE|EE_(?:CURVE_TYPE_(?:ANSI_X9_62|DEFAULT|MONTGOMERY|WEIERSTRASS)|PRIME_TYPE_(?:DEFAULT|FEE|GENERAL|MERSENNE))|IELDVALUE_COMPLEX_DATA_TYPE)|HINT_(?:ADDRESS_(?:APP|SP)|NONE)|INVALID_HANDLE|K(?:EY(?:ATTR_(?:ALWAYS_SENSITIVE|EXTRACTABLE|MODIFIABLE|NEVER_EXTRACTABLE|P(?:ARTIAL|ERMANENT|RIVATE|UBLIC_KEY_ENCRYPT)|RETURN_(?:D(?:ATA|EFAULT)|NONE|REF)|SENSITIVE)|BLOB_(?:OTHER|R(?:AW(?:_FORMAT_(?:BSAFE|CCA|FIPS186|MSCAPI|NONE|O(?:CTET_STRING|PENSS(?:H(?:2)?|L)|THER)|P(?:GP|KCS(?:1|3|8))|SPKI|VENDOR_DEFINED|X509))?|EF(?:ERENCE|_FORMAT_(?:INTEGER|OTHER|S(?:PKI|TRING))))|WRAPPED(?:_FORMAT_(?:APPLE_CUSTOM|MSCAPI|NONE|O(?:PENSS(?:H1|L)|THER)|PKCS(?:7|8)))?)|CLASS_(?:OTHER|P(?:RIVATE_KEY|UBLIC_KEY)|SE(?:CRET_PART|SSION_KEY))|HEADER_VERSION|USE_(?:ANY|DE(?:CRYPT|RIVE)|ENCRYPT|SIGN(?:_RECOVER)?|UNWRAP|VERIFY(?:_RECOVER)?|WRAP)|_HIERARCHY_(?:EXPORT|INTEG|NONE))|R_(?:BASE_ERROR|PRIVATE_ERROR))|LIST_(?:ELEMENT_(?:DATUM|SUBLIST|WORDID)|TYPE_(?:CUSTOM|SEXPR|UNKNOWN))|M(?:DS_(?:BASE_ERROR|PRIVATE_ERROR)|ODULE_STRING_SIZE)|N(?:ET_PROTO_(?:C(?:MP(?:S)?|USTOM)|FTP(?:S)?|LDAP(?:NS|S)?|NONE|OCSP|UNSPECIFIED|X500DAP)|OTIFY_(?:FAULT|INSERT|REMOVE))|OK|P(?:ADDING_(?:A(?:LTERNATE|PPLE_SSLv2)|C(?:IPHERSTEALING|USTOM)|FF|NONE|ONE|PKCS(?:1|5|7)|RANDOM|SIGRAW|VENDOR_DEFINED|ZERO)|KCS(?:5_PBKDF2_PRF_HMAC_SHA1|_OAEP_(?:MGF(?:1_(?:MD5|SHA1)|_NONE)|PSOURCE_(?:NONE|Pspecified)))|RIVILEGE_SCOPE_(?:NONE|PROCESS|THREAD)|VC_(?:APP|NONE|SP))|QUERY_(?:RETURN_DATA|SIZELIMIT_NONE|TIMELIMIT_NONE)|S(?:AMPLE_TYPE_(?:ASYMMETRIC_KEY|BIOMETRIC|COMMENT|HASHED_PASSWORD|KEY(?:BAG_KEY|CHAIN_(?:CHANGE_LOCK|LOCK|PROMPT))|P(?:ASSWORD|R(?:EAUTH|O(?:CESS|MPTED_(?:BIOMETRIC|PASSWORD)|TECTED_(?:BIOMETRIC|PASSWORD))))|RETRY_ID|S(?:IGNED_(?:NONCE|SECRET)|YMMETRIC_KEY)|THRESHOLD)|ERVICE_(?:AC|C(?:L|S(?:P|SM))|DL|KR|TP))|T(?:P_(?:A(?:CTION_(?:ALLOW_EXPIRED(?:_ROOT)?|CRL_SUFFICIENT|DEFAULT|FETCH_C(?:ERT_FROM_NET|RL_FROM_NET)|IMPLICIT_ANCHORS|LEAF_IS_CA|REQUIRE_(?:CRL_(?:IF_PRESENT|PER_CERT)|REV_PER_CERT)|TRUST_SETTINGS)|UTHORITY_REQUEST_C(?:ERT(?:ISSUE|NOTARIZE|RE(?:SUME|VOKE)|SUSPEND|USERECOVER|VERIFY)|RLISSUE))|BASE_(?:ERROR|TP_ERROR)|C(?:ERT(?:CHANGE_(?:HOLD|NO(?:NE|T_AUTHORIZED)|OK(?:WITHNEWTIME)?|RE(?:ASON_(?:AFFILIATIONCHANGE|C(?:ACOMPROMISE|EASEOPERATION)|HOLDRELEASE|KEYCOMPROMISE|SU(?:PERCEDED|SPECTEDCOMPROMISE)|UNKNOWN)|JECTED|LEASE|VOKE)|STATUS_UNKNOWN|WRONGCA)|ISSUE_(?:NOT_AUTHORIZED|OK(?:WITH(?:CERTMODS|SERVICEMODS))?|REJECTED|STATUS_UNKNOWN|WILL_BE_REVOKED)|NOTARIZE_(?:NOT_AUTHORIZED|OK(?:WITH(?:OUTFIELDS|SERVICEMODS))?|REJECTED|STATUS_UNKNOWN)|RECLAIM_(?:NO(?:MATCH|T_AUTHORIZED)|OK|REJECTED|STATUS_UNKNOWN)|VERIFY_(?:EXPIRED|INVALID(?:_(?:AUTHORITY|BASIC_CONSTRAINTS|C(?:ERT(?:GROUP|_VALUE)|RL_DIST_PT)|NAME_TREE|POLICY(?:_IDS)?|SIGNATURE))?|NOT_VALID_YET|REVOKED|SUSPENDED|UNKNOWN(?:_CRITICAL_EXT)?|VALID)|_(?:DIR_UPDATE|NOTIFY_RENEW|PUBLISH))|ONFIRM_(?:ACCEPT|REJECT|STATUS_UNKNOWN)|RL(?:ISSUE_(?:INVALID_DOMAIN|NOT_(?:AUTHORIZED|CURRENT)|OK|REJECTED|STATUS_UNKNOWN|UNKNOWN_IDENTIFIER)|_DISTRIBUTE))|FORM_TYPE_(?:GENERIC|REGISTRATION)|KEY_ARCHIVE|PRIVATE_ERROR|STOP_ON_(?:FIRST_(?:FAIL|PASS)|NONE|POLICY))|RUE)|USEE_(?:AUTHENTICATION|DOMESTIC|FINANCIAL|INSURANCE|K(?:EYEXCH|R(?:ENT|LE))|LAST|MEDICAL|NONE|SSL|WEAK)|VALUE_NOT_AVAILABLE|WORDID_(?:A(?:CL|LPHA|SYMMETRIC_KEY)?|B(?:ER|I(?:NARY|OMETRIC))?|C(?:ANCELED|ERT|OMMENT|RL|USTOM)?|D(?:ATE|B(?:S_(?:CREATE|DELETE)|_(?:DELETE|EXEC_STORED_QUERY|INSERT|MODIFY|READ))|E(?:CRYPT|L(?:ETE|TA_CRL)|R(?:IVE)?)|ISPLAY|O|SA(?:_SHA1)?)?|E(?:LGAMAL|N(?:CRYPT|TRY)|XPORT_(?:CLEAR|WRAPPED))?|G(?:E(?:NKEY)?)?|HA(?:SH(?:ED_(?:PASSWORD|SUBJECT))?|VAL)|I(?:BCHASH|MPORT_(?:CLEAR|WRAPPED)|NTEL|SSUER(?:_INFO)?)|K(?:E(?:A|Y(?:BAG_KEY|CHAIN_(?:CHANGE_LOCK|LOCK|PROMPT)|HOLDER)?)|_OF_N)|L(?:E|OGIN(?:_NAME)?)?|M(?:AC|D(?:2(?:WITHRSA)?|4|5(?:WITHRSA)?))|N(?:AME|DR|HASH|OT_(?:AFTER|BEFORE)|U(?:LL|MERIC))?|O(?:BJECT_HASH|N(?:E_TIME|LINE)|WNER)|P(?:A(?:M_NAME|RTITION|SSWORD)|GP|IN|R(?:E(?:AUTH(?:_SOURCE)?|FIX)|IVATE_KEY|O(?:CESS|MPTED_(?:BIOMETRIC|PASSWORD)|PAGATE|TECTED_(?:BIOMETRIC|P(?:ASSWORD|IN))))|UBLIC_KEY(?:_FROM_CERT)?)?|Q|R(?:ANGE|EVAL|IPEM(?:AC|D(?:160)?)|SA(?:_(?:ISO9796|PKCS(?:1(?:_(?:MD5|S(?:HA1|IG)))?|_(?:MD5|SHA1))?|RAW))?)|S(?:DSIV1|E(?:QUENCE|T|XPR)|HA1(?:WITH(?:DSA|ECDSA|RSA))?|IGN(?:ATURE|ED_(?:NONCE|SECRET))?|PKI|UBJECT(?:_INFO)?|Y(?:MMETRIC_KEY|STEM))|T(?:AG|HRESHOLD|IME)|URI|VE(?:NDOR_(?:END|START)|RSION)|X(?:509(?:V(?:1|2|3)|_ATTRIBUTE)|9_ATTRIBUTE)|_(?:FIRST_UNUSED|NLU_|RESERVED_1|STAR_|UNK_))|X509_DATAFORMAT_(?:ENCODED|PA(?:IR|RSED))))|_MAX_PATH)|antDecompress|o(?:mm(?:entID|onID)|pyrightID))|D(?:RAWHook|T_(?:Authority(?:InfoAccess|KeyID)|BasicConstraints|C(?:ertPolicies|rl(?:DistributionPoints|Number|Reason))|DeltaCrl|ExtendedKeyUsage|I(?:nhibitAnyPolicy|ssu(?:erAltName|ingDistributionPoint))|KeyUsage|N(?:ameConstraints|etscapeCertType)|Other|Policy(?:Constraints|Mappings)|QC_Statements|Subject(?:AltName|KeyID)))|E(?:OLHook|QUALTO|V(?:HIDE|LEVEL|MOVE|NOP|SHOW))|F(?:ORMID|or(?:matVersionID|ward(?:BackwardLooping|Looping)))|G(?:NT_(?:D(?:NSName|irectoryName)|EdiPartyName|IPAddress|OtherName|R(?:FC822Name|egisteredID)|URI|X400Address)|REATERTHAN)|H(?:DActivity|ITTESTHook)|I(?:dleActivity|nstrumentID)|KAEISHandleCGI|L(?:A(?:TENCY_QOS_TIER_(?:0|1|2|3|4|5|UNSPECIFIED)|UNCH_DATA_(?:ARRAY|BOOL|DICTIONARY|ERRNO|FD|INTEGER|MACHPORT|OPAQUE|REAL|STRING))|ESSTHAN)|M(?:ACE(?:3Type|6Type)|IDIDataID|PLibrary_(?:DevelopmentRevision|M(?:ajorVersion|inorVersion)|Release)|arkerID)|N(?:X_(?:BigEndian|L(?:eftButton|ittleEndian)|OneButton|RightButton|UnknownByteOrder)|ameID|etActivity|o(?:Looping|neType))|O(?:S(?:A(?:ControlFlowError|Duplicate(?:Handler|P(?:arameter|roperty))|I(?:llegal(?:A(?:ccess|ssign)|Index|Range)|nconsistentDeclarations)|M(?:essageNotUnderstood|issingParameter)|ParameterMismatch|Syntax(?:Error|TypeError)|TokenTooLong|Undefined(?:Handler|Variable))|_LOG_TYPE_(?:DE(?:BUG|FAULT)|ERROR|FAULT|INFO))|verallAct)|S(?:SL_(?:DH(?:E_(?:DSS_(?:EXPORT_WITH_DES40_CBC_SHA|WITH_(?:3DES_EDE_CBC_SHA|DES_CBC_SHA))|RSA_(?:EXPORT_WITH_DES40_CBC_SHA|WITH_(?:3DES_EDE_CBC_SHA|DES_CBC_SHA)))|_(?:DSS_(?:EXPORT_WITH_DES40_CBC_SHA|WITH_(?:3DES_EDE_CBC_SHA|DES_CBC_SHA))|RSA_(?:EXPORT_WITH_DES40_CBC_SHA|WITH_(?:3DES_EDE_CBC_SHA|DES_CBC_SHA))|anon_(?:EXPORT_WITH_(?:DES40_CBC_SHA|RC4_40_MD5)|WITH_(?:3DES_EDE_CBC_SHA|DES_CBC_SHA|RC4_128_MD5))))|FORTEZZA_DMS_WITH_(?:FORTEZZA_CBC_SHA|NULL_SHA)|N(?:O_SUCH_CIPHERSUITE|ULL_WITH_NULL_NULL)|RSA_(?:EXPORT_WITH_(?:DES40_CBC_SHA|RC(?:2_CBC_40_MD5|4_40_MD5))|WITH_(?:3DES_EDE_CBC_(?:MD5|SHA)|DES_CBC_(?:MD5|SHA)|IDEA_CBC_(?:MD5|SHA)|NULL_(?:MD5|SHA)|RC(?:2_CBC_MD5|4_128_(?:MD5|SHA)))))|lpTypeErr|oundDataID)|T(?:ASK_(?:BACKGROUND_APPLICATION|CONTROL_APPLICATION|D(?:ARWINBG_APPLICATION|EFAULT_APPLICATION)|FOREGROUND_APPLICATION|GRAPHICS_SERVER|INSPECT_BASIC_COUNTS|NONUI_APPLICATION|RENICED|THROTTLE_APPLICATION|UNSPECIFIED)|HROUGHPUT_QOS_TIER_(?:0|1|2|3|4|5|UNSPECIFIED)|LS_(?:AES_(?:128_(?:CCM_(?:8_SHA256|SHA256)|GCM_SHA256)|256_GCM_SHA384)|CHACHA20_POLY1305_SHA256|DH(?:E_(?:DSS_WITH_(?:3DES_EDE_CBC_SHA|AES_(?:128_(?:CBC_SHA(?:256)?|GCM_SHA256)|256_(?:CBC_SHA(?:256)?|GCM_SHA384)))|PSK_WITH_(?:3DES_EDE_CBC_SHA|AES_(?:128_(?:CBC_SHA(?:256)?|GCM_SHA256)|256_(?:CBC_SHA(?:384)?|GCM_SHA384))|NULL_SHA(?:256|384)?|RC4_128_SHA)|RSA_WITH_(?:3DES_EDE_CBC_SHA|AES_(?:128_(?:CBC_SHA(?:256)?|GCM_SHA256)|256_(?:CBC_SHA(?:256)?|GCM_SHA384))))|_(?:DSS_WITH_(?:3DES_EDE_CBC_SHA|AES_(?:128_(?:CBC_SHA(?:256)?|GCM_SHA256)|256_(?:CBC_SHA(?:256)?|GCM_SHA384)))|RSA_WITH_(?:3DES_EDE_CBC_SHA|AES_(?:128_(?:CBC_SHA(?:256)?|GCM_SHA256)|256_(?:CBC_SHA(?:256)?|GCM_SHA384)))|anon_WITH_(?:3DES_EDE_CBC_SHA|AES_(?:128_(?:CBC_SHA(?:256)?|GCM_SHA256)|256_(?:CBC_SHA(?:256)?|GCM_SHA384))|RC4_128_MD5)))|E(?:CDH(?:E_(?:ECDSA_WITH_(?:3DES_EDE_CBC_SHA|AES_(?:128_(?:CBC_SHA(?:256)?|GCM_SHA256)|256_(?:CBC_SHA(?:384)?|GCM_SHA384))|CHACHA20_POLY1305_SHA256|NULL_SHA|RC4_128_SHA)|PSK_WITH_AES_(?:128_CBC_SHA|256_CBC_SHA)|RSA_WITH_(?:3DES_EDE_CBC_SHA|AES_(?:128_(?:CBC_SHA(?:256)?|GCM_SHA256)|256_(?:CBC_SHA(?:384)?|GCM_SHA384))|CHACHA20_POLY1305_SHA256|NULL_SHA|RC4_128_SHA))|_(?:ECDSA_WITH_(?:3DES_EDE_CBC_SHA|AES_(?:128_(?:CBC_SHA(?:256)?|GCM_SHA256)|256_(?:CBC_SHA(?:384)?|GCM_SHA384))|NULL_SHA|RC4_128_SHA)|RSA_WITH_(?:3DES_EDE_CBC_SHA|AES_(?:128_(?:CBC_SHA(?:256)?|GCM_SHA256)|256_(?:CBC_SHA(?:384)?|GCM_SHA384))|NULL_SHA|RC4_128_SHA)|anon_WITH_(?:3DES_EDE_CBC_SHA|AES_(?:128_CBC_SHA|256_CBC_SHA)|NULL_SHA|RC4_128_SHA)))|MPTY_RENEGOTIATION_INFO_SCSV)|NULL_WITH_NULL_NULL|PSK_WITH_(?:3DES_EDE_CBC_SHA|AES_(?:128_(?:CBC_SHA(?:256)?|GCM_SHA256)|256_(?:CBC_SHA(?:384)?|GCM_SHA384))|CHACHA20_POLY1305_SHA256|NULL_SHA(?:256|384)?|RC4_128_SHA)|RSA_(?:PSK_WITH_(?:3DES_EDE_CBC_SHA|AES_(?:128_(?:CBC_SHA(?:256)?|GCM_SHA256)|256_(?:CBC_SHA(?:384)?|GCM_SHA384))|NULL_SHA(?:256|384)?|RC4_128_SHA)|WITH_(?:3DES_EDE_CBC_SHA|AES_(?:128_(?:CBC_SHA(?:256)?|GCM_SHA256)|256_(?:CBC_SHA(?:256)?|GCM_SHA384))|NULL_(?:MD5|SHA(?:256)?)|RC4_128_(?:MD5|SHA))))|extWidthHook)|U(?:NORDERED|srActivity)|W(?:DEFNFnd|IDTHHook)|XPC_ACTIVITY_STATE_(?:C(?:HECK_IN|ONTINUE)|D(?:EFER|ONE)|RUN|WAIT)|a(?:b(?:brevDate|ortErr)|c(?:tiv(?:Dev|Mask|ateEvt|eFlag(?:Bit)?)|uteUpr(?:A|I|O|U))|d(?:bAddrMask|d(?:Re(?:fFailed|sFailed)|Size(?:Bit)?))|eBuildSyntax(?:Bad(?:D(?:ata|esc)|EOF|Hex|Negative|Token)|CoercedList|MissingQuote|No(?:C(?:lose(?:Brac(?:e|ket)|Hex|Paren|String)|olon)|E(?:OF|rr)|Key)|OddHex|Uncoerced(?:DoubleAt|Hex))|fp(?:A(?:ccessDenied|lready(?:LoggedInErr|Mounted)|uthContinue)|B(?:ad(?:DirIDType|IDErr|UAM|VersNum)|itmapErr)|C(?:a(?:llNot(?:Allowed|Supported)|nt(?:Mo(?:untMoreSrvre|ve)|Rename)|talogChanged)|ontainsSharedErr)|D(?:enyConflict|i(?:ffVolErr|rNot(?:Empty|Found)|skFull))|EofError|F(?:ileBusy|latVol)|I(?:D(?:Exists|NotFound)|conTypeError|nside(?:SharedErr|TrashErr)|temNotFound)|LockErr|MiscErr|No(?:MoreLocks|Server)|Object(?:Exists|Locked|NotFound|TypeErr)|P(?:armErr|wd(?:ExpiredErr|NeedsChangeErr|PolicyErr|SameErr|TooShortErr))|Range(?:NotLocked|Overlap)|S(?:ame(?:NodeErr|ObjectErr)|e(?:rverGoingDown|ssClosed))|TooManyFilesOpen|UserNotAuth|VolLocked)|l(?:phaLock(?:Bit)?|tDBoxProc)|pp(?:1(?:Evt|Mask)|2(?:Evt|Mask)|3(?:Evt|Mask)|4(?:Evt|Mask)|IsDaemon|M(?:emFullErr|odeErr)|VersionTooOld|e(?:arance(?:Bad(?:BrushIndexErr|CursorIndexErr|TextColorIndexErr)|Process(?:NotRegisteredErr|RegisteredErr)|ThemeHasNoAccents)|ndDITL(?:Bottom|Right))|le(?:Logo|MenuFolderIconResource))|s(?:i(?:AliasName|ParentName|ServerName|VolumeName|ZoneName)|p(?:B(?:adVersNum|ufTooSmall)|No(?:Ack|MoreSess|Servers)|ParamErr|S(?:e(?:rverBusy|ssClosed)|izeErr)|TooMany))|t(?:AbsoluteCenter|Bottom(?:Left|Right)?|Center(?:Bottom|Left|Right|Top)|HorizontalCenter|Left|None|Right|Top(?:Left|Right)?|VerticalCenter|om(?:IndexInvalidErr|sNotOfSameTypeErr)|p(?:BadRsp|LenErr))|u(?:t(?:hFailErr|oKey(?:Mask)?)|xiliaryExportDataUnavailable))|b(?:A(?:ccessCntl|llowCDiDataHandler|ncestorModDateChanges)|DoNotDisplay|Ha(?:ndleAERecording|s(?:B(?:TreeMgr|lankAccessPrivileges)|C(?:atSearch|opyFile)|D(?:esktopMgr|irectIO)|ExtFSVol|F(?:ileIDs|olderLock)|MoveRename|OpenDeny|PersonalAccessPrivileges|ShortName|UserGroupList))|Is(?:AutoMounted|Case(?:Preserving|Sensitive)|Ejectable|On(?:ExternalBus|InternalBus)|Removable)|L(?:2PCanMapFileBlocks|anguageMask|imitFCBs|ocalWList)|No(?:BootBlks|DeskItems|LclSync|MiniFndr|RootTimes|S(?:witchTo|ysDir)|V(?:NEdit|olumeSizes))|ParentModDateChanges|S(?:cript(?:LanguageMask|Mask)|upports(?:2TBFiles|AsyncRequests|Ex(?:clusiveLocks|tendedFileSecurity)|FS(?:CatalogSearch|ExchangeObjects)|HFSPlusAPIs|Journaling|LongNames|MultiScriptNames|NamedForks|S(?:ubtreeIterators|ymbolicLinks)|TrashVolumeCache))|T(?:akeActiveEvent|rshOffLine)|a(?:d(?:ATPSkt|B(?:tSlpErr|uffNum)|C(?:allOrderErr|hannel|ksmErr|o(?:decCharacterizationErr|mponent(?:Instance|Selector|Type)|ntrollerHeight))|D(?:BtSlp|Cksum|ataRefIndex|e(?:lim|pthErr)|ictFormat|rag(?:FlavorErr|ItemErr|RefErr))|E(?:dit(?:Index|List|ionFileErr)|nding|xtResource)|F(?:CBErr|i(?:dErr|leFormat)|o(?:lderDescErr|rmat))|I(?:mage(?:Description|Err|RgnErr)|nputText)|LocNameErr|M(?:DBErr|ovErr)|P(?:asteboard(?:FlavorErr|I(?:ndexErr|temErr)|SyncErr)|ortNameErr|rofileError|ublicMovieAtom)|R(?:eqErr|outingSizeErr)|S(?:GChannel|crapRefErr|e(?:ctionErr|rviceMethodErr)|ubPartErr)|Tra(?:ckIndex|nslation(?:RefErr|SpecErr))|UnitErr)|se(?:DblQuote|SingQuote))|dNamErr|re(?:akRecd|veMark)|t(?:DupRecErr|Key(?:AttrErr|LenErr)|NoSpace|RecNotFnd|n(?:Ctrl|State(?:Bit)?))|uf(?:2SmallErr|TooSmall|fer(?:IsSmall|sTooSmall)))|c(?:A(?:DBAddress|EList|ccessory(?:Process|Suitcase)|ddress(?:Spec)?|lias(?:File|List|OrString)|p(?:pl(?:eTalkAddress|ication(?:File|Process)?)|ril)|rc|ugust)|B(?:o(?:dyColor|olean)|usAddress)|C(?:ell|har|l(?:assIdentifier|ipping(?:File|Window)|osure)|o(?:erc(?:e(?:KataHiragana|LowerCase|OneByteToTwoByte|Remove(?:Diacriticals|Hyphens|Punctuation|WhiteSpace)|SmallKana|UpperCase|Zenkakuhankaku)|ion)|l(?:orTable|umn)|n(?:stant|t(?:ainer(?:Window)?|entSpace|rolPanelFile))))|D(?:TPWindow|e(?:cember|pthErr|sk(?:AccessoryFile|top(?:Printer)?)|v(?:Err|Spec))|isk|ocument(?:File)?|rawingArea|ynamicLibrary)|E(?:n(?:tireContents|umeration)|thernetAddress|ventIdentifier)|F(?:TPItem|ebruary|i(?:le|reWireAddress|xed(?:Point|Rectangle)?)|o(?:lder|nt(?:File|Suitcase))|r(?:ame(?:Color|work)|iday))|Gr(?:aphic(?:Line|Object|Shape|Text)|oup(?:edGraphic)?)|H(?:TML|andle(?:Breakpoint|r))|I(?:PAddress|conFamily|n(?:foWindow|sertion(?:Loc|Point)|t(?:ern(?:alFinderObject|etAddress)|l(?:Text|WritingCode)))|tem)|J(?:anuary|u(?:ly|ne))|Key(?:Form|Identifier|stroke)|L(?:abel|i(?:n(?:e|kedList)|st(?:Element|Or(?:Record|String)|RecordOrString)?)|o(?:calTalkAddress|ng(?:DateTime|Fixed(?:Point|Rectangle)?|Integer|Point|Rectangle)))|M(?:a(?:chine(?:Loc)?|rch|tchErr|y)|enu(?:Item)?|issingValue|on(?:day|th))|N(?:o(?:MemErr|vember)|umber(?:DateTimeOrString|Or(?:DateTime|String))?)|O(?:bject(?:BeingExamined|Specifier)?|ctober|nline(?:Disk|LocalDisk|RemoteDisk)|penableObject|val)|P(?:ICT|a(?:ckage|ragraph)|ixel(?:Map)?|olygon|r(?:e(?:ferences(?:Window)?|position)|o(?:ce(?:dure|ss)|perty|tectErr)))|QD(?:Point|Rectangle)|R(?:GBColor|a(?:ngeErr|wData)|e(?:al|c(?:ord|tangle)|ference|sErr)|o(?:tation|undedRectangle|w)|unningAddress)|S(?:CSIAddress|aturday|cript(?:ingAddition)?|e(?:conds|lection|ptember)|h(?:ar(?:ableContainer|ing(?:Privileges|Window))|ortInteger)|mallReal|ound(?:File)?|pecialFolders|t(?:atusWindow|orage|ring(?:Class)?)|u(?:itcase|nday)|ymbol)|T(?:able|e(?:mpMemErr|xt(?:Color|Flow|Styles)?)|hu(?:mbColor|rsday)|okenRingAddress|rash|uesday|ype)|U(?:RL|SBAddress|ndefined|ser(?:Identifier)?)|Ve(?:ctor|rsion)|W(?:e(?:dnesday|ekday)|indow|ord|ritingCodeInfo)|Zone|a(?:l(?:Arabic(?:Civil|Lunar)|Coptic|Gregorian|J(?:apanese|ewish)|Persian|l(?:NotSupportedByNodeErr|erSecuritySession))|n(?:cel|not(?:BeLeafAtomErr|DeferErr|FindAtomErr|M(?:akeContiguousErr|oveAttachedController)|SetWidthOfAttachedController)|t(?:Create(?:PickerWindow|SingleForkFile)|DoThatInCurrentMode|EnableTrack|FindHandler|GetFlavorErr|LoadP(?:ackage|ick(?:MethodErr|er))|OpenHandler|PutPublicMovieAtom|Re(?:adUtilities|ceiveFromSynthesizerOSErr)|S(?:endToSynthesizerOSErr|tepErr)))|tChangedErr|utionIcon)|bNotFound|dev(?:GenErr|MemErr|ResErr|Unset)|e(?:dilla|nt(?:eredDot|ury))|frag(?:A(?:bortClosureErr|rchitectureErr)|C(?:F(?:M(?:InternalErr|StartupErr)|ragRsrcErr)|losureIDErr|on(?:nectionIDErr|t(?:ainerIDErr|extIDErr)))|DupRegistrationErr|ExecFileRefErr|F(?:i(?:leSizeErr|rst(?:ErrCode|ReservedCode))|ragment(?:CorruptErr|FormatErr|UsageErr))|I(?:mportToo(?:NewErr|OldErr)|nit(?:AtBootErr|FunctionErr|LoopErr|OrderErr))|L(?:astErrCode|ibConnErr)|MapFileErr|No(?:ApplicationErr|ClientMemErr|IDsErr|LibraryErr|P(?:ositionErr|rivateMemErr)|RegistrationErr|S(?:ectionErr|ymbolErr)|tClosureErr)|OutputLengthErr|R(?:eservedCode_(?:1|2|3)|srcForkErr)|StdFolderErr|UnresolvedErr)|h(?:a(?:nnel(?:Busy|NotBusy)|rCodeMask)|eckBoxProc|kCtrl)|ircumflex(?:Upr(?:A|E|I|O|U))?|kSumErr|l(?:earDev|k(?:RdErr|WrErr)|os(?:Err|eDev)|rBit)|m(?:1(?:0CLRData|1CLRData|2CLRData|3CLRData|4CLRData|5CLRData|6_8ColorPacking)|24_8ColorPacking|3(?:2_(?:16ColorPacking|32ColorPacking|8ColorPacking)|CLRData)|4(?:0_8ColorPacking|8_(?:16ColorPacking|8ColorPacking)|CLRData)|5(?:6_8ColorPacking|CLRData)|6(?:4_(?:16ColorPacking|8ColorPacking)|CLRData)|7CLRData|8(?:CLRData|_8ColorPacking)|9CLRData|A(?:RGB(?:32(?:PmulSpace|Space)|64(?:L(?:PmulSpace|Space)|PmulSpace|Space))|ToB(?:0Tag|1Tag|2Tag)|b(?:ortWriteAccess|s(?:oluteColorimetric|tractClass))|lpha(?:FirstPacking|LastPacking|PmulSpace|Space)|sciiData)|B(?:ToA(?:0Tag|1Tag|2Tag)|e(?:ginAccess|stMode)|inaryData|l(?:ackPointCompensation(?:Mask)?|ue(?:ColorantTag|TRCTag))|radfordChromaticAdaptation|ufferBasedProfile)|C(?:M(?:SReservedFlagsMask|Y(?:Data|K(?:32Space|64(?:LSpace|Space)|Data|Space)))|S(?:1(?:C(?:hromTag|ustTag)|NameTag|ProfileVersion|TRCTag)|2ProfileVersion)|a(?:librationDateTimeTag|meraDeviceClass|nt(?:Co(?:ncatenateError|pyModifiedV1Profile)|Delete(?:Element|Profile)|GamutCheckError|XYZ))|h(?:arTargetTag|romaticAdaptationTag)|lose(?:Access|Spool)|o(?:lorSpace(?:AlphaMask|Class|EncodingMask|P(?:ackingMask|remulAlphaMask)|ReservedMask|Space(?:AndAlphaMask|Mask))|pyrightTag)|reateNewAccess|urrent(?:DeviceInfoVersion|Profile(?:InfoVersion|LocationSize|MajorVersion)))|D(?:e(?:fault(?:DeviceID|ProfileID)|vice(?:AlreadyRegistered|DBNotFoundErr|InfoVersion1|M(?:fgDescTag|odelDescTag)|NotRegistered|Profile(?:InfoVersion(?:1|2)|sNotFound)|State(?:AppleRsvdBits|Busy|De(?:fault|viceRsvdBits)|ForceNotify|Offline)))|isplay(?:Class|DeviceClass|Use)|raftMode)|E(?:lementTagNotFound|mbedded(?:Mask|Profile|Use(?:Mask)?)|ndAccess|rrIncompatibleProfile)|F(?:atalProfileErr|lare(?:0|100))|G(?:amut(?:CheckingMask|Result(?:1Space|Space)|Tag)|eometry(?:0(?:45or450|dord0)|Unknown)|lossy(?:MatteMask)?|r(?:ay(?:16(?:LSpace|Space)|8Space|A(?:16(?:PmulSpace|Space)|32(?:L(?:PmulSpace|Space)|PmulSpace|Space)|PmulSpace|Space)|Data|Space|TRCTag)|een(?:ColorantTag|TRCTag)))|H(?:LS(?:32Space|Data|Space)|SV(?:32Space|Data|Space))|I(?:CC(?:ProfileVersion(?:2(?:1)?|4)|ReservedFlagsMask)|lluminant(?:A|D(?:5(?:0|5)|65|93)|EquiPower|F(?:2|8)|Unknown)|n(?:dexRangeErr|put(?:Class|Use)|ter(?:nalCFErr|polationMask)|valid(?:ColorSpace|DstMap|Profile(?:Comment|Location)?|S(?:earch|rcMap)))|terate(?:AllDeviceProfiles|Cu(?:rrentDeviceProfiles|stomDeviceProfiles)|DeviceProfilesMask|FactoryDeviceProfiles))|L(?:AB(?:24Space|32Space|48(?:LSpace|Space)|Space)|UV(?:32Space|Space)|abData|i(?:n(?:e(?:arChromaticAdaptation|sPer)|kClass)|ttleEndianPacking)|ong(?:10ColorPacking|8ColorPacking)|u(?:minanceTag|vData))|M(?:C(?:Eight(?:8Space|Space)|Five(?:8Space|Space)|H(?:5Data|6Data|7Data|8Data)|S(?:even(?:8Space|Space)|ix(?:8Space|Space)))|a(?:cintosh|gicNumber|keAndModelTag)|e(?:asurementTag|dia(?:BlackPointTag|WhitePointTag)|thod(?:Error|NotFound))|icrosoft)|N(?:a(?:med(?:Color(?:2Tag|Class|NotFound|Tag)|Data|Indexed(?:32(?:LSpace|Space)|Space))|tiveDisplayInfoTag)|o(?:C(?:olorPacking|urrentProfile)|GDevicesError|ProfileBase|Space|rmalMode)|umHeaderElements)|O(?:neBitDirectPacking|pen(?:Read(?:Access|Spool)|Write(?:Access|Spool))|riginalProfileLocationSize|utput(?:Class|Use))|P(?:S(?:2(?:C(?:RD(?:0Tag|1Tag|2Tag|3Tag|VMSizeTag)|SATag)|RenderingIntentTag)|7bit|8bit)|a(?:rametricType(?:0|1|2|3|4)|thBasedProfile)|erceptual|r(?:e(?:fsSynchError|view(?:0Tag|1Tag|2Tag))|interDeviceClass|o(?:file(?:Description(?:MLTag|Tag)|Error|IterateDataVersion(?:1|2|3|4)|MajorVersionMask|NotFound|SequenceDescTag|sIdentical)|of(?:DeviceClass|Use))|trDefaultScreens))|QualityMask|R(?:GB(?:16(?:LSpace|Space)|24Space|32Space|48(?:LSpace|Space)|565(?:LSpace|Space)|A(?:32(?:PmulSpace|Space)|64(?:L(?:PmulSpace|Space)|PmulSpace|Space)|PmulSpace|Space)|Data|Space)|angeOverFlow|e(?:ad(?:Access|Spool)|d(?:ColorantTag|TRCTag)|flective(?:TransparentMask)?|lativeColorimetric|servedSpace(?:1|2)|verseChannelPacking))|S(?:RGB(?:16ChannelEncoding|Data)|aturation|c(?:annerDeviceClass|reening(?:DescTag|Tag))|earchError|i(?:g(?:C(?:rdInfoType|urveType)|Dat(?:aType|eTimeType)|Lut(?:16Type|8Type)|M(?:akeAndModelType|easurementType|ulti(?:Funct(?:A2BType|B2AType)|LocalizedUniCodeType))|Na(?:medColor(?:2Type|Type)|tiveDisplayInfoType)|P(?:S2CRDVMSizeType|arametricCurveType|rofile(?:DescriptionType|SequenceDescType))|S(?:15Fixed16Type|creeningType|ignatureType)|TextType|U(?:1(?:6Fixed16Type|Fixed15Type)|Int(?:16Type|32Type|64Type|8Type)|crBgType|nicodeTextType)|Vi(?:deoCardGammaType|ewingConditionsType)|XYZType)|liconGraphics)|olaris|potFunction(?:Cross|D(?:efault|iamond)|Ellipse|Line|Round|Square|Unknown)|tdobs(?:19(?:31TwoDegrees|64TenDegrees)|Unknown))|T(?:aligent|echnology(?:AMDisplay|CRTDisplay|D(?:igitalCamera|yeSublimationPrinter)|Electro(?:photographicPrinter|staticPrinter)|F(?:ilm(?:Scanner|Writer)|lexography)|Gravure|InkJetPrinter|OffsetLithography|P(?:MDisplay|hoto(?:CD|ImageSetter|graphicPaperPrinter)|rojectionTelevision)|ReflectiveScanner|Silkscreen|T(?:ag|hermalWaxPrinter)|Video(?:Camera|Monitor)))|U(?:crBgTag|nsupportedDataType|seDefaultChromaticAdaptation)|V(?:i(?:deoCardGamma(?:FormulaType|Ta(?:bleType|g))|ewingConditions(?:DescTag|Tag))|onKriesChromaticAdaptation)|W(?:ord5(?:65ColorPacking|ColorPacking)|rite(?:Access|Spool))|XYZ(?:24Space|32Space|48(?:LSpace|Space)|Data|Space)|Y(?:CbCrData|XY(?:32Space|Space)|xyData)|apFontTableTag|dKey(?:Bit)?|p(?:Alias(?:NoFlags|OnlyThisFile)|IsMissing|ThreadSafe|WantsRegisterMessage))|o(?:dec(?:AbortErr|BadDataErr|C(?:ant(?:QueueErr|WhenErr)|onditionErr)|D(?:ataVersErr|isabledErr|roppedFrameErr)|E(?:rr|xtensionNotFoundErr)|ImageBufErr|N(?:eed(?:AccessKeyErr|ToFlushChainErr)|o(?:MemoryPleaseWaitErr|thingToBlitErr))|O(?:ffscreenFailed(?:Err|PleaseRetryErr)|penErr)|ParameterDialogConfirm|S(?:creenBufErr|izeErr|poolErr)|UnimpErr|WouldOffscreenErr)|l(?:lection(?:I(?:ndexRangeErr|tem(?:LockedErr|NotFoundErr))|VersionErr)|or(?:SyncNotInstalled|sRequestedErr))|mponent(?:AutoVersionIncludeFlags|D(?:ll(?:EntryNotFoundErr|LoadErr)|o(?:AutoVersion|ntRegister))|HasMultiplePlatforms|LoadResident|Not(?:Captured|ThreadSafeErr)|WantsUnregister)|n(?:nectionInvalid|straintReachedErr|t(?:ainer(?:AlreadyOpenWrn|NotFoundWrn)|rol(?:Err|HandleInvalidErr|InvalidDataVersionErr|Key(?:Bit)?|P(?:anelFolderIconResource|roperty(?:Invalid|NotFoundErr))|ler(?:BoundsNotExact|HasFixedHeight))))|pyDev|r(?:Err|eFoundationUnknownErr)|uld(?:Not(?:ParseSourceFileErr|ResolveDataRef|UseAnExistingSample)|ntGetRequiredComponent))|rash|trlItem|u(?:r(?:NumberPartsVersion|r(?:LeadingZ|NegSym|SymLead|TrailingZ|ent(?:CurLang|DefLang))|sorDev)|tDev))|d(?:BoxProc|InstErr|RemovErr|a(?:t(?:a(?:Already(?:Closed|OpenForWrite)|No(?:DataRef|tOpenFor(?:Read|Write))|VerErr)|e(?:StdMask|Time(?:Invalid|NotFound)))|y(?:Field|LdingZ|Mask|Of(?:Week(?:Field|Mask)|Year(?:Field|Mask))))|blDagger|c(?:eExtErr|m(?:B(?:ad(?:D(?:ataSizeErr|ictionaryErr)|F(?:eatureErr|i(?:eld(?:InfoErr|TypeErr)|ndMethodErr))|KeyErr|PropertyErr)|lockFullErr|ufferOverflowErr)|D(?:ictionary(?:BusyErr|NotOpenErr)|upRecordErr)|IterationCompleteErr|N(?:ecessaryFieldErr|o(?:AccessMethodErr|FieldErr|RecordErr|tDictionaryErr))|P(?:aramErr|ermissionErr|rotectedErr)|TooManyKeyErr))|dp(?:LenErr|SktErr)|e(?:activDev|bugging(?:Duplicate(?:OptionErr|SignatureErr)|ExecutionContextErr|Invalid(?:NameErr|OptionErr|SignatureErr)|No(?:CallbackErr|MatchErr))|fault(?:Component(?:Any(?:Flags(?:AnyManufacturer(?:AnySubType)?)?|Manufacturer|SubType)|Identical)|PhysicalEntryCount)|limPad|s(?:criptorFontTableTag|k(?:PatID|top(?:DamagedErr|IconResource))|tPortErr)|viceCantMeetRequest)|i(?:a(?:eresisUpr(?:E|I|Y)|log(?:Kind|NoTimeoutErr))|ffVolErr|giUnimpErr|r(?:FulErr|NFErr|ectXObjectAlreadyExists)|sk(?:Evt|Mask))|my|o(?:All|Color|F(?:ace|ont)|Size|Toggle|cumentProc|m(?:Cannot|Native|TranslateFirst|Wildcard)|tlessLwrI|ubleAcute)|r(?:agNotAcceptedErr|iver(?:Evt|HardwareGoneErr|Mask)|opFolderIconResource|vQType)|s(?:32BitMode|AddressErr|B(?:ad(?:L(?:aunch|ibrary)|Patch(?:Header)?|S(?:ANEOpcode|lotInt|tartupDisk))|u(?:fPtrTooLow|sError))|C(?:DEFNotFound|antHoldSystemHeap|hkErr|oreErr)|Di(?:rtyDisk|sassemblerInstalled)|ExtensionsDisabled|F(?:PErr|SErr|inderErr|orcedQuit)|G(?:ibblyMovedToDisabledFolder|reeting)|H(?:D20Installed|MenuFindErr)|I(?:OCoreErr|llInstErr|rqErr)|L(?:ine(?:AErr|FErr)|o(?:adErr|stConnectionToNetworkDisk))|M(?:B(?:ATA(?:PISysError|SysError)|ExternFlpySysError|FlpySysError|SysError|arNFnd)|DEFNotFound|ac(?:OSROMVersionTooOld|sBugInstalled)|emFullErr|i(?:scErr|xedModeFailure)|ustUseFCBAccessors)|N(?:eedToWriteBootBlocks|o(?:Exts(?:Disassembler|MacsBug)|FPU|P(?:a(?:ckErr|tch)|k(?:1|2|3|4|5|6|7))|t(?:EnoughRAMToBoot|The1)))|O(?:ldSystem|vflowErr)|P(?:CCardATASysError|arityErr|rivErr)|R(?:AMDiskTooBig|e(?:insert|moveDisk))|S(?:CSIWarn|hutDownOrRes(?:tart|ume)|tknHeap|witchOffOrRestart|ys(?:Err|tem(?:FileErr|RequiresPowerPC)))|TraceErr|UnBootableSystem|VM(?:BadBackingStore|DeferredFuncTableFull)|W(?:DEFNotFound|riteToSupervisorStackGuardPage)|ZeroDivErr|kFulErr)|tQType|u(?:mmyType|p(?:FNErr|licate(?:AtomTypeAndIDErr|F(?:lavorErr|olderDescErr)|HandlerErr|PasteboardFlavorErr|RoutingErr|ScrapFlavorErr))|ration(?:Day|Forever|Hour|Mi(?:crosecond|llisecond|nute)|NoWait|Second))|ym)|e(?:A(?:DB|ddressSpec|nalogAudio|ppleTalk|udio(?:Line(?:In|Out)|Out))|Bus|C(?:DROM|apsLockDown|learKey|o(?:mm(?:Slot|andDown)|n(?:duit|trolDown)))|D(?:VD|e(?:leteKey|viceType)|i(?:gitalAudio|splay)|ownArrowKey)|E(?:n(?:dKey|terKey)|scapeKey|thernet)|F(?:1(?:0Key|1Key|2Key|3Key|4Key|5Key|Key)|2Key|3Key|4Key|5Key|6Key|7Key|8Key|9Key|ireWire|loppy|orwardDelKey)|H(?:D|elpKey|omeKey)|I(?:P|RTalk|nfrared|rDA)|Key(?:Kind|board)|L(?:CD|e(?:ftArrowKey|nErr)|ocalTalk)|M(?:ac(?:IP|Video)|icrophone|o(?:d(?:em(?:P(?:ort|rinterPort))?|ifiers)|nitorOut|use)|ultiErr)|NuBus(?:Card)?|OptionDown|P(?:C(?:I(?:bus|card)|card)|DS(?:card|slot)|PP|age(?:DownKey|UpKey)|o(?:intingDevice|stScript)|r(?:inter(?:Port)?|otocol))|R(?:eturnKey|ightArrowKey)|S(?:CSI|VGA|cheme|erial|hiftDown|peakers|torageDevice|video)|T(?:abKey|okenRing|rack(?:ball|pad))|U(?:SB|pArrowKey)|Video(?:In|Monitor|Out)|WS(?:ArrayType|BooleanType|D(?:at(?:aType|eType)|ictionaryType|oubleType)|IntegerType|NullType|StringType|UnknownType)|dit(?:Text|i(?:ngNotAllowed|onMgrInitErr))|mptyPathErr|n(?:dOfDataReached|um(?:A(?:fterDate|l(?:iases|l(?:D(?:isks|ocuments)|LocalDisks|OpenFolders|RemoteDisks))|nyDate|rr(?:angement|ows))|B(?:e(?:foreDate|tweenDate)|ooleanValues)|Con(?:flicts|sid(?:erations|sAndIgnores))|Date|ExistingItems|Fo(?:lders|ntsPanel)|Ge(?:neralPanel|stalt)|I(?:conSize|nfoWindowPanel)|Justification|KeyForm|La(?:rgeIconSize|st(?:Month|Week|Year))|M(?:emoryPanel|i(?:niIconSize|scValues))|O(?:lderItems|nDate)|P(?:osition|r(?:efs(?:ButtonViewPanel|GeneralPanel|IconViewPanel|L(?:abelPanel|istViewPanel)|WindowPanel)|otection))|Quality|S(?:aveOptions|haringPanel|mallIconSize|ortDirection(?:Normal|Reverse)?|t(?:at(?:ionery|usNConfigPanel)|yle))|T(?:his(?:Month|Week|Year)|oday|ransferMode)|ViewBy|Where|Yesterday)|v(?:BadVers|NotPresent|VersTooBig))|ofErr|r(?:a(?:Field|Mask)|r(?:A(?:E(?:AccessorNotFound|B(?:ad(?:KeyForm|ListItem|TestKey)|u(?:fferTooSmall|ildSyntaxError))|C(?:ant(?:HandleClass|PutThatThere|SupplyType|Undo)|o(?:ercionFail|rruptData))|D(?:esc(?:IsNull|NotFound)|uplicateHandler)|E(?:mptyListContainer|vent(?:F(?:ailed|iltered)|Not(?:Handled|Permitted)|WouldRequireUserConsent))|HandlerNotFound|I(?:llegalIndex|mpossibleRange|n(?:Transaction|dexTooLarge))|LocalOnly|N(?:e(?:gativeCount|werVersion)|o(?:Such(?:Logical|Object|Transaction)|User(?:Interaction|Selection)|t(?:A(?:EDesc|S(?:ingleObject|pecialFunction)|n(?:E(?:lement|numMember)|ObjSpec)|ppleEvent)|Modifiable)))|P(?:aramMissed|r(?:ivilegeError|opertiesClash))|Re(?:adDenied|c(?:eive(?:EscapeCurrent|Terminate)|ordingIsAlreadyOn)|plyNot(?:Arrived|Valid))|Stream(?:AlreadyConverted|BadNesting)|T(?:argetAddressNotPermitted|imeout|ypeError)|Unknown(?:AddressType|ObjectType|SendMode)|ValueOutOfRange|W(?:aitCanceled|r(?:iteDenied|ong(?:DataType|NumberArgs))))|S(?:CantCo(?:mpareMoreThan32k|nsiderAndIgnore)|I(?:llegalFormalParameter|nconsistentNames)|NoResultReturned|ParameterNotForEvent|TerminologyNestingTooDeep)|borted|lreadyInImagingMode|ttention|uthorization(?:BadAddress|Canceled|Denied|ExternalizeNotAllowed|In(?:ter(?:actionNotAllowed|nal(?:izeNotAllowed)?)|valid(?:Flags|Pointer|Ref|Set|Tag))|Success|ToolE(?:nvironmentError|xecuteFailure)))|C(?:an(?:NotInsertWhileWalkProcInProgress|notUndo|tEmbed(?:IntoSelf|Root))|o(?:ntrol(?:DoesntSupportFocus|HiddenOrDisabled|IsNotEmbedder|sAlreadyExist)|r(?:eEndianData(?:DoesNotMatchFormat|Too(?:LongForFormat|ShortForFormat))|ruptWindowDescription)|uldntSetFocus)|pp(?:General|Last(?:SystemDefinedError|UserDefinedError)|bad_(?:alloc|cast|exception|typeid)|domain_error|i(?:nvalid_argument|os_base_failure)|l(?:ength_error|ogic_error)|o(?:ut_of_range|verflow_error)|r(?:ange_error|untime_error)|underflow_error))|D(?:SPQueueSize|ata(?:Browser(?:I(?:nvalidProperty(?:Data|Part)|temNot(?:Added|Found))|NotConfigured|PropertyNot(?:Found|Supported))|NotSupported|SizeMismatch))|E(?:mptyScrap|n(?:dOf(?:Body|Document)|gineNotFound))|F(?:S(?:AttributeNotFound|Bad(?:AllocFlags|Buffer|F(?:SRef|ork(?:Name|Ref))|I(?:nfoBitmap|te(?:mCount|ratorFlags))|PosMode|SearchParams)|Fork(?:Exists|NotFound)|IteratorNot(?:Found|Supported)|Missing(?:CatInfo|Name)|N(?:ameTooLong|o(?:MoreItems|t(?:AFolder|EnoughSpaceForOperation)))|OperationNotSupported|PropertyNotValid|QuotaExceeded|RefsDifferent|UnknownCall)|inder(?:AppFolderProtected|B(?:adPackageContents|oundsWrong)|C(?:an(?:notPutAway|t(?:DeleteImmediately|Move(?:Source|To(?:Ancestor|Destination))|Overwrite|UseTrashedItems))|orruptOpenFolderList)|FileSharingMustBeOn|I(?:ncestuousMove|sBusy|temAlreadyInDest)|L(?:astReserved|ockedItemsInTrash)|MustBeActive|NoInvisibleFiles|OnlyLockedItemsInTrash|Pro(?:gramLinkingMustBeOn|perty(?:DoesNotApply|NowWindowBased))|S(?:harePointsCantInherit|ysFolderProtected)|Un(?:knownUser|supportedInsidePackages)|VolumeNotFound|Window(?:MustBe(?:ButtonView|IconView|ListView)|NotOpen|WrongType))|loatingWindowsNotInitialized|wdReset)|HMIllegalContentForM(?:aximumState|inimumState)|I(?:A(?:AllocationErr|BufferTooSmall|Canceled|EndOfTextRun|InvalidDocument|No(?:Err|MoreItems)|ParamErr|TextExtractionErr|UnknownErr)|nvalid(?:PartCode|Range|Window(?:P(?:roperty|tr)|Ref))|te(?:m(?:AlreadyInTree|Not(?:Control|FoundInTree))|ratorReachedEnd))|K(?:B(?:Fail(?:Setting(?:ID|TranslationTable)|WritePreference)|IlligalParameters|PS2KeyboardNotAvailable)|C(?:AuthFailed|BufferTooSmall|CreateChainFailed|D(?:ata(?:Not(?:Available|Modifiable)|TooLarge)|uplicate(?:Callback|Item|Keychain))|I(?:n(?:teraction(?:NotAllowed|Required)|valid(?:Callback|ItemRef|Keychain|SearchRef))|temNotFound)|KeySizeNotAllowed|No(?:CertificateModule|DefaultKeychain|PolicyModule|S(?:torageModule|uch(?:Attr|Class|Keychain))|tAvailable)|ReadOnly(?:Attr)?|WrongKCVersion))|M(?:arginWilllNotFit|essageNotSupported)|N(?:eedsCompositedWindow|o(?:HiliteText|RootControl|nContiuousAttribute|t(?:InImagingMode|ValidTree)))|O(?:SA(?:AppNotHighLevelEventAware|BadS(?:elector|torageType)|C(?:ant(?:A(?:ccess|ssign)|C(?:oerce|reate)|GetTerminology|Launch|OpenComponent|StorePointers)|o(?:mponentMismatch|rrupt(?:Data|Terminology)))|D(?:ata(?:BlockTooLarge|Format(?:Obsolete|TooNew))|ivideByZero)|GeneralError|In(?:ternalTableOverflow|validID)|N(?:oSuchDialect|umericOverflow)|RecordingIsAlreadyOn|S(?:criptError|ourceNotAvailable|tackOverflow|ystemError)|TypeError)|ffset(?:I(?:nvalid|sOutsideOfView)|NotOnElementBounday)|pen(?:Denied|ing))|R(?:e(?:adOnlyText|fNum)|ootAlreadyExists)|S(?:SL(?:ATS(?:C(?:ertificate(?:HashAlgorithmViolation|TrustViolation)|iphersuiteViolation)|LeafCertificateHashAlgorithmViolation|Minimum(?:KeySizeViolation|VersionViolation)|Violation)|B(?:ad(?:C(?:ert(?:ificateStatusResponse)?|ipherSuite|onfiguration)|RecordMac)|ufferOverflow)|C(?:ert(?:Expired|NotYetValid|ificateRequired)|l(?:ient(?:CertRequested|HelloReceived)|osed(?:Abort|Graceful|NoNotify))|on(?:figurationFailed|nectionRefused)|rypto)|Dec(?:o(?:deError|mpressFail)|ryptionFail)|FatalAlert|H(?:andshakeFail|ostNameMismatch)|I(?:llegalParam|n(?:appropriateFallback|ternal))|M(?:issingExtension|oduleAttach)|N(?:e(?:gotiation|tworkTimeout)|oRootCert)|P(?:eer(?:A(?:ccessDenied|uthCompleted)|Bad(?:Cert|RecordMac)|Cert(?:Expired|Revoked|Unknown)|Dec(?:o(?:deError|mpressFail)|rypt(?:Error|ionFail))|ExportRestriction|HandshakeFail|In(?:sufficientSecurity|ternalError)|NoRenegotiation|ProtocolVersion|RecordOverflow|U(?:n(?:expectedMsg|knownCA|supportedCert)|serCancelled))|rotocol)|RecordOverflow|SessionNotFound|TransportReset|Un(?:expected(?:Message|Record)|known(?:PSKIdentity|RootCert)|recognizedName|supportedExtension)|W(?:eakPeerEphemeralDHKey|ouldBlock)|XCertChainInvalid)|e(?:c(?:A(?:CL(?:AddFailed|ChangeFailed|DeleteFailed|NotSimple|ReplaceFailed)|ddin(?:LoadFailed|UnloadFailed)|l(?:gorithmMismatch|locate|readyLoggedIn)|pple(?:AddAppACLSubject|InvalidKey(?:EndDate|StartDate)|PublicKeyIncomplete|S(?:SLv2Rollback|ignatureMismatch))|tt(?:achHandleBusy|ributeNotInContext)|uthFailed)|B(?:adReq|lockSizeMismatch|ufferTooSmall)|C(?:RL(?:AlreadySigned|BadURI|Expired|Not(?:Found|Trusted|ValidYet)|PolicyFailed|ServerDown)|S(?:AmbiguousBundleFormat|Bad(?:BundleFormat|CallbackValue|Di(?:ctionaryFormat|skImageFormat)|FrameworkVersion|LVArch|MainExecutable|NestedCode|ObjectFormat|Resource|TeamIdentifier)|C(?:MSTooLarge|ancelled)|D(?:B(?:Access|Denied)|SStoreSymlink|bCorrupt)|FileHardQuarantined|GuestInvalid|H(?:elperFailed|ost(?:Protocol(?:Contradiction|DedicationError|Invalid(?:Attribute|Hash)|NotProxy|RelativePath|StateError|Unrelated)|Reject))|In(?:foPlistFailed|ternalError|valid(?:A(?:ssociatedFileData|ttributeValues)|Entitlements|Flags|ObjectRef|Platform|RuntimeVersion|Symlink|TeamIdentifier))|MultipleGuests|No(?:Ma(?:inExecutable|tches)|SuchCode|t(?:A(?:Host|ppLike)|Supported))|O(?:bjectRequired|utdated)|Re(?:gularFile|q(?:Failed|Invalid|Unsupported)|source(?:DirectoryFailed|NotSupported|RulesInvalid|s(?:Invalid|Not(?:Found|Sealed)))|vokedNotarization)|S(?:ig(?:DB(?:Access|Denied)|nature(?:Failed|Invalid|NotVerifiable|Un(?:supported|trusted)))|taticCode(?:Changed|NotFound))|TooBig|Un(?:implemented|s(?:ealed(?:AppRoot|FrameworkRoot)|igned(?:NestedCode)?|upported(?:DigestAlgorithm|GuestAttributes)))|Vetoed|WeakResource(?:Envelope|Rules))|allbackFailed|ertificate(?:CannotOperate|Expired|N(?:ameNotAllowed|otValidYet)|PolicyNotAllowed|Revoked|Suspended|ValidityPeriodTooLong)|o(?:deSigning(?:Bad(?:CertChainLength|PathLengthConstraint)|Development|No(?:BasicConstraints|ExtendedKeyUsage))|nversionError|reFoundationUnknown)|reateChainFailed)|D(?:ata(?:Not(?:Available|Modifiable)|TooLarge|baseLocked|storeIsOpen)|e(?:code|vice(?:Error|Failed|Reset|VerifyFailed))|iskFull|skFull|uplicate(?:Callback|Item|Keychain))|E(?:MM(?:LoadFailed|UnloadFailed)|ndOfData|ventNotificationCallbackNotFound|xtendedKeyUsageNotCritical)|F(?:i(?:eldSpecifiedMultiple|leTooBig)|unction(?:Failed|IntegrityFail))|HostNameMismatch|I(?:DPFailure|O|n(?:DarkWake|comp(?:atible(?:DatabaseBlob|FieldFormat|KeyBlob|Version)|leteCertRevocationCheck)|putLengthError|sufficientC(?:lientID|redentials)|ter(?:action(?:NotAllowed|Required)|nal(?:Component|Error))|val(?:dCRLAuthority|id(?:A(?:CL|c(?:cess(?:Credentials|Request)|tion)|ddinFunctionTable|lgorithm(?:Parms)?|ttribute(?:AccessCredentials|B(?:ase|lockSize)|DLDBHandle|E(?:ffectiveBits|ndDate)|I(?:nitVector|terationCount)|Key(?:Length|Type)?|Label|Mode|OutputSize|P(?:a(?:dding|ssphrase)|ri(?:me|vateKeyFormat)|ublicKeyFormat)|R(?:andom|ounds)|S(?:alt|eed|tartDate|ubprime|ymmetricKeyFormat)|Version|WrappedKeyFormat)|uthority(?:KeyID)?)|B(?:aseACLs|undleInfo)|C(?:RL(?:Encoding|Group|Index|Type)?|allback|ert(?:Authority|ificate(?:Group|Ref))|ontext)|D(?:BL(?:ist|ocation)|ata(?:baseBlob)?|igestAlgorithm)|E(?:ncoding|xtendedKeyUsage)|FormType|GUID|Handle(?:Usage)?|I(?:D(?:Linkage)?|dentifier|n(?:dex(?:Info)?|putVector)|temRef)|Key(?:AttributeMask|Blob|Format|Hierarchy|Label|Ref|Usage(?:ForPolicy|Mask)|chain)|LoginName|ModifyMode|N(?:ame|e(?:tworkAddress|wOwner)|umberOfFields)|O(?:utputVector|wnerEdit)|P(?:VC|a(?:rsingModule|ss(?:throughID|wordRef))|o(?:inter|licyIdentifiers)|refsDomain)|Query|R(?:e(?:ason|cord|quest(?:Inputs|or)|sponseVector)|oot)|S(?:ampleValue|cope|e(?:archRef|rviceMask)|ignature|topOnPolicy|ub(?:ServiceID|ject(?:KeyID|Name)))|T(?:imeString|rustSetting(?:s)?|uple(?:Credendtials|Group)?)|Val(?:idityPeriod|ue))))|temNotFound)|Key(?:BlobTypeIncorrect|HeaderInconsistent|IsSensitive|SizeNotAllowed|UsageIncorrect)|LibraryReferenceNotFound|M(?:DSError|emoryError|issing(?:A(?:lgorithmParms|ttribute(?:AccessCredentials|B(?:ase|lockSize)|DLDBHandle|E(?:ffectiveBits|ndDate)|I(?:nitVector|terationCount)|Key(?:Length|Type)?|Label|Mode|OutputSize|P(?:a(?:dding|ssphrase)|ri(?:me|vateKeyFormat)|ublicKeyFormat)|R(?:andom|ounds)|S(?:alt|eed|tartDate|ubprime|ymmetricKeyFormat)|Version|WrappedKeyFormat))|Entitlement|RequiredExtension|Value)|o(?:bileMe(?:CSRVerifyFailure|FailedConsistencyCheck|NoRequestPending|Request(?:AlreadyPending|Queued|Redirected)|Server(?:AlreadyExists|Error|NotAvailable|ServiceErr))|dule(?:Man(?:ager(?:InitializeFailed|NotFound)|ifestVerifyFailed)|NotLoaded))|ultiple(?:ExecSegments|PrivKeys|ValuesUnsupported))|N(?:etworkFailure|o(?:AccessForItem|BasicConstraints(?:CA)?|CertificateModule|Default(?:Authority|Keychain)|FieldValues|PolicyModule|S(?:torageModule|uch(?:Attr|Class|Keychain))|TrustSettings|t(?:Available|Initialized|LoggedIn|Signer|Trusted)))|O(?:CSP(?:BadRe(?:quest|sponse)|No(?:Signer|tTrustedToAnchor)|Respon(?:der(?:InternalError|MalformedReq|SignatureRequired|TryLater|Unauthorized)|seNonceMismatch)|S(?:ignatureError|tatusUnrecognized)|Unavailable)|pWr|utputLengthError)|P(?:VC(?:AlreadyConfigured|ReferentNotFound)|a(?:ram|ssphraseRequired|thLengthConstraintExceeded)|kcs12VerifyFailure|olicyNotFound|rivilegeNot(?:Granted|Supported)|ublicKeyInconsistent)|Qu(?:erySizeUnknown|otaExceeded)|Re(?:adOnly(?:Attr)?|cordModified|jectedForm|quest(?:Descriptor|Lost|Rejected)|sourceSignBad(?:CertChainLength|ExtKeyUsage))|S(?:MIME(?:Bad(?:ExtendedKeyUsage|KeyUsage)|EmailAddressesNotFound|KeyUsageNotCritical|NoEmailAddress|SubjAltNameNotCritical)|SLBadExtendedKeyUsage|e(?:lfCheckFailed|rviceNotAvailable)|igningTimeMissing|tagedOperation(?:InProgress|NotStarted)|uccess)|T(?:agNotFound|imestamp(?:AddInfoNotAvailable|Bad(?:Alg|DataFormat|Request)|Invalid|Missing|NotTrusted|Re(?:jection|vocation(?:Notification|Warning))|S(?:erviceNotAvailable|ystemFailure)|TimeNotAvailable|Unaccepted(?:Extension|Policy)|Waiting)|rust(?:NotAvailable|SettingDeny))|U(?:n(?:implemented|known(?:C(?:RLExtension|ertExtension|riticalExtensionFlag)|Format|QualifiedCertStatement|Tag)|supported(?:AddressType|F(?:ieldFormat|ormat)|IndexInfo|Key(?:AttributeMask|Format|Label|Size|UsageMask)|Locality|Num(?:Attributes|Indexes|RecordTypes|SelectionPreds)|Operator|QueryLimits|Service|VectorOfBuffers))|serCanceled)|Verif(?:icationFailure|y(?:ActionFailed|Failed))|Wr(?:Perm|ongSecVersion))|ssion(?:AuthorizationDenied|In(?:ternal|valid(?:Attributes|Flags|Id))|Success|ValueNotSet))|tate)|T(?:askNotFound|opOf(?:Body|Document)|reeIsLocked)|U(?:n(?:known(?:AttributeTag|Control|Element)|recognizedWindowClass|supportedWindowAttributesForClass)|serWantsToDragWindow)|W(?:S(?:InternalError|ParseError|T(?:imeoutError|ransportError))|indow(?:Does(?:Not(?:FitOnscreen|HaveProxy)|ntSupportFocus)|NotFound|PropertyNotFound|RegionCodeInvalid|sAlreadyInitialized))))|url(?:A(?:FP|T)|EPPC|F(?:TP|ile)|Gopher|HTTP(?:S)?|IMAP|L(?:DAP|aunch)|M(?:ail(?:box)?|essage|ulti)|N(?:FS|NTP|ews)|POP|RTSP|SNews|Telnet|Unknown)|v(?:Type|e(?:nt(?:AlreadyPostedErr|ClassIn(?:correctErr|validErr)|DeferAccessibilityEventErr|H(?:andlerAlreadyInstalledErr|otKey(?:ExistsErr|InvalidErr))|InternalErr|KindIncorrectErr|Loop(?:QuitErr|TimedOutErr)|Not(?:HandledErr|InQueueErr)|Pa(?:rameterNotFoundErr|ssToNextTargetErr)|TargetBusyErr)|ryEvent)|tNotEnb)|x(?:UserBreak|cessCollsns|t(?:FSErr|en(?:dedBlock(?:Len)?|sionsFolderIconResource)|ra(?:ctErr|neousStrings))))|f(?:B(?:adPartsTable|estGuess|syErr)|D(?:esktop|isk)|E(?:mptyFormatString|xtra(?:Decimal|Exp|Percent|Separator))|Form(?:StrIsNAN|atO(?:K|verflow))|HasBundle|Invisible|LckdErr|Missing(?:Delimiter|Literal)|Negative|O(?:nDesk|utOfSynch)|Positive|SpuriousChars|Trash|VNumber|Zero|a(?:ceBit|talDateTime)|e(?:ature(?:FontTableTag|Unsupported)|tchReference)|i(?:Ligature|d(?:Exists|NotFound)|eldOrderNotIntl|le(?:BoundsErr|OffsetTooBigErr)|rst(?:DskErr|PickerError))|l(?:Ligature|avor(?:DataPromised|NotSaved|S(?:ender(?:Only|Translated)|ystemTranslated)|Type(?:HFS|PromiseHFS))|o(?:at(?:GrowProc|Proc|Side(?:GrowProc|Proc|Zoom(?:GrowProc|Proc))|Zoom(?:GrowProc|Proc))|ppyIconResource))|mt(?:1Err|2Err)|n(?:OpnErr|fErr)|o(?:nt(?:Bit|DecError|Not(?:Declared|OutlineErr)|Panel(?:FontSelectionQDStyleVersionErr|S(?:electionStyleErr|howErr))|SubErr|sFolderIconResource)|r(?:ceRead(?:Bit|Mask)|m(?:A(?:bsolutePosition|lias)|Creator|Name|PropertyID|R(?:ange|elativePosition)|Test|U(?:niqueID|serPropertyID)|Whose)))|raction|s(?:AtMark|CurPerm|D(?:SIntErr|ataTooBigErr)|From(?:LEOF|Mark|Start)|QType|R(?:d(?:AccessPerm|DenyPerm|Perm|Wr(?:Perm|ShPerm))|nErr|t(?:DirID|ParID))|SB(?:A(?:ccessDate(?:Bit)?|ttributeModDate(?:Bit)?)|Dr(?:BkDat(?:Bit)?|CrDat(?:Bit)?|FndrInfo(?:Bit)?|MdDat(?:Bit)?|NmFls(?:Bit)?|ParID(?:Bit)?|UsrWds(?:Bit)?)|F(?:l(?:Attrib(?:Bit)?|BkDat(?:Bit)?|CrDat(?:Bit)?|FndrInfo(?:Bit)?|LgLen(?:Bit)?|MdDat(?:Bit)?|P(?:arID(?:Bit)?|yLen(?:Bit)?)|R(?:LgLen(?:Bit)?|PyLen(?:Bit)?)|XFndrInfo(?:Bit)?)|ullName(?:Bit)?)|GroupID(?:Bit)?|N(?:egate(?:Bit)?|odeID(?:Bit)?)|P(?:artialName(?:Bit)?|ermissions(?:Bit)?)|Skip(?:HiddenItems(?:Bit)?|PackageContents(?:Bit)?)|UserID(?:Bit)?)|UnixPriv|Wr(?:AccessPerm|DenyPerm|Perm)|m(?:B(?:adF(?:FSNameErr|SD(?:LenErr|VersionErr))|usyFFSErr)|DuplicateFSIDErr|FFSNotFoundErr|NoAlternateStackErr|UnknownFSMMessageErr))|ullTrashIconResource)|g(?:WorldsNotSameDepthAndSizeErr|crOnMFMErr|e(?:n(?:CdevRangeBit|eric(?:ApplicationIconResource|CDROMIconResource|D(?:eskAccessoryIconResource|ocumentIconResource)|E(?:ditionFileIconResource|xtensionIconResource)|F(?:ileServerIconResource|olderIconResource)|HardDiskIconResource|MoverObjectIconResource|PreferencesIconResource|QueryDocumentIconResource|RAMDiskIconResource|S(?:tationeryIconResource|uitcaseIconResource)))|stalt(?:16Bit(?:AudioSupport|SoundIO)|20thAnniversary|32Bit(?:Addressing|Capable|QD(?:1(?:1|2|3))?|SysZone)|68(?:0(?:00|10|20|30(?:MMU)?|40(?:FPU|MMU)?)|8(?:51|8(?:1|2))|k)|8BitQD|A(?:DB(?:ISOKbdII|KbdII)|FPClient(?:3_(?:5|6(?:_(?:1|2|3))?|7(?:_2)?|8(?:_(?:1|3|4))?)|AttributeMask|CfgRsrc|MultiReq|SupportsIP|V(?:MUI|ersionMask))?|LM(?:Attr|Has(?:CFMSupport|RescanNotifiers|SF(?:Group|Location))|Present|Vers)|MU|T(?:A(?:Attr|Present)|SU(?:AscentDescentControlsFeature|B(?:atchBreakLinesFeature|iDiCursorPositionFeature|yCharacterClusterFeature)|D(?:ecimalTabFeature|irectAccess|ropShadowStyleFeature)|F(?:allbacks(?:Feature|ObjFeatures)|eatures)|GlyphBoundsFeature|Highlight(?:ColorControlFeature|InactiveTextFeature)|IgnoreLeadingFeature|L(?:ayoutC(?:acheClearFeature|reateAndCopyFeature)|ineControlFeature|owLevelOrigFeatures)|MemoryFeature|NearestCharLineBreakFeature|PositionToCursorFeature|StrikeThroughStyleFeature|T(?:abSupportFeature|extLocatorUsageFeature|rackingFeature)|U(?:nderlineOptionsStyleFeature|pdate(?:1|2|3|4|5|6|7))|Version)|alkVersion)|UXVersion|VLTree(?:Attr|PresentBit|Supports(?:HandleBasedTreeBit|TreeLockingBit))|WS(?:6150_6(?:0|6)|8(?:150_(?:110|80)|550)|9150_(?:120|80))|d(?:dressingModeAttr|minFeaturesFlagsAttr)|l(?:iasMgr(?:Attr|FollowsAliasesWhenResolving|Pre(?:fersPath|sent)|Re(?:quiresAccessors|solveAliasFileWithMountOptions)|Supports(?:AOCEKeychain|ExtendedCalls|FSCalls|RemoteAppletalk))|legroQD(?:Text)?|tivecRegistersSwappedCorrectlyBit)|ntiAliasedTextAvailable|pp(?:earance(?:Attr|CompatMode|Exists|Version)|le(?:Adjust(?:ADBKbd|ISOKbd|Keypad)|Events(?:Attr|Present)|Guide(?:IsDebug|Present)|Script(?:Attr|P(?:owerPCSupport|resent)|Version)|TalkVersion))|rbitorAttr|syncSCSI(?:INROM)?)|Bu(?:iltInSoundInput|sClkSpeed(?:MHz)?)|C(?:FM(?:99Present(?:Mask)?|Attr|Present(?:Mask)?)|PU(?:486|6(?:0(?:1|3(?:e(?:v)?)?|4(?:e(?:v)?)?)|80(?:00|10|20|30|40))|750(?:FX)?|970(?:FX|MP)?|Apollo|G4(?:74(?:47|50))?|Pentium(?:4|II|Pro)?|X86)|RM(?:Attr|P(?:ersistentFix|resent)|ToolRsrcCalls)|TBVersion|a(?:n(?:StartDragInFloatWindow|UseCGTextRendering)|r(?:bonVersion|dServicesPresent))|l(?:assic(?:II)?|oseView(?:Attr|DisplayMgrFriendly|Enabled))|o(?:l(?:lectionMgrVersion|or(?:Matching(?:Attr|LibLoaded|Version)|Picker(?:Version)?|Sync(?:1(?:0(?:4|5)?|1)|2(?:0|1(?:1|2|3)?|5|6(?:1)?)|30)))|mp(?:onent(?:Mgr|Platform)|ressionMgr)|n(?:nMgr(?:Attr|CMSearchFix|ErrorString|MultiAsyncIO|Present)|t(?:extualMenu(?:Attr|Has(?:AttributeAndModifierKeys|UnicodeSupport)|TrapAvailable|UnusedBit)|rol(?:M(?:gr(?:Attr|Present(?:Bit)?|Version)|sgPresentMask)|Strip(?:Attr|Exists|User(?:Font|HotKey)|Version(?:Fixed)?))))|untOfCPUs)|reatesAliasFontRsrc|urrentGraphicsVersion)|D(?:BAccessMgr(?:Attr|Present)|ITLExt(?:Attr|Present|SupportsIctb)|T(?:MgrSupportsFSM|P(?:Features|Info))|esktop(?:Pictures(?:Attr|Displayed|Installed)|SpeechRecognition)|i(?:alogM(?:gr(?:Attr|HasAquaAlert(?:Bit|Mask)|Present(?:Bit|Mask)?)|sgPresentMask)|ctionaryMgr(?:Attr|Present)|gitalSignatureVersion|s(?:kCacheSize|playMgr(?:Attr|C(?:an(?:Confirm|SwitchMirrored)|olorSyncAware)|GeneratesProfiles|Present|S(?:etDepthNotifies|leepNotifies)|Vers)))|ra(?:gMgr(?:Attr|FloatingWind|HasImageSupport|Present)|wSprocketVersion)|upSelectorErr)|E(?:MMU1|asyAccess(?:Attr|Locked|O(?:ff|n)|Sticky)|ditionMgr(?:Attr|Present|TranslationAware)|xt(?:ADBKbd|ISOADBKbd|ToolboxTable|en(?:ded(?:TimeMgr|WindowAttributes(?:Bit|Mask)?)|sionTableVersion)))|F(?:BC(?:CurrentVersion|IndexingState|Version|indexing(?:Critical|Safe))|PUType|S(?:A(?:llowsConcurrentAsyncIO|ttr)|IncompatibleDFA82|M(?:DoesDynamicLoad|Version)|NoMFSVols|Supports(?:2TBVols|4GBVols|DirectIO|ExclusiveLocks|H(?:FSPlusVols|ardLinkDetection))|UsesPOSIXPathsForConversion)|XfrMgr(?:A(?:sync|ttr)|ErrorString|MultiFile|Present)|i(?:le(?:AllocationZeroedBlocksBit|Mapping(?:Attr|MultipleFilesFix|Present))|nd(?:Folder(?:Attr|Present|RedirectionAttr)|er(?:Attr|CallsAEProcess|DropEvent|F(?:loppyRootComments|ullDragManagerSupport)|HasClippings|LargeAndNotSavedFlavorsOK|MagicPlacement|Supports4GBVolumes|U(?:nderstandsRedirectedDesktopFolder|ses(?:ExtensibleFolderManager|SpecialOpenFoldersFile))))|rstSlotNumber)|loppy(?:Attr|IsM(?:FMOnly|anualEject)|UsesDiskInPlace)|o(?:lder(?:DescSupport|Mgr(?:FollowsAliasesWhenResolving|Supports(?:Domains|ExtendedCalls|FSCalls)))|ntMgrAttr)|rontWindowMayBeHidden(?:Bit|Mask)|ullExtFSDispatching)|G(?:X(?:PrintingMgrVersion|Version)|raphics(?:Attr|Is(?:Debugging|Loaded|PowerPC)|Version))|H(?:a(?:rdware(?:Attr|Vendor(?:Apple|Code))|s(?:ASC|Color|D(?:eepGWorlds|irectPixMaps)|E(?:nhancedLtalk|xtendedDiskInit)|F(?:MTuner|SSpecCalls|ileSystemManager|loatingWindows(?:Bit|Mask)?)|G(?:PI(?:aTo(?:DCDa|RTxCa)|bToDCDb)|rayishTextOr)|H(?:FSPlusAPIs|WClosedCaptioning)|IRRemote|ParityCapability|ResourceOverrides|S(?:C(?:C|SI(?:96(?:1|2))?)|erialFader|ingleWindowMode(?:Bit|Mask)|o(?:ftPowerOff|und(?:Fader|InputDevice))|tereoDecoder|ystemIRFunction)|TVTuner|UniversalROM|V(?:IA(?:1|2)|idDecoderScaler)|Window(?:Buffering(?:Bit|Mask)?|Shadows(?:Bit|Mask))|ZoomedVideo))|elpMgr(?:Attr|Extensions|Present)|i(?:dePort(?:A|B)|ghLevelMatching))|I(?:NeedIRPowerOffConfirm|PCSupport|RDisabled|conUtilities(?:Attr|Has(?:32BitIcons|48PixelIcons|8BitDeepMasks|IconServices)|Present)|n(?:itHeapZerosOutHeapsBit|te(?:l|rnalDisplay)))|JapanAdjustADBKbd|K(?:BPS2(?:Keyboards|Set(?:IDToAny|TranslationTable))|eyboard(?:Type|sAttr))|L(?:aunch(?:C(?:anReturn|ontrol)|FullFileSpec)|ineLevelInput|o(?:cationErr|gical(?:PageSize|RAMSize)|wMemorySize))|M(?:B(?:Legacy|MultipleBays|SingleBay)|MUType|P(?:CallableAPIsAttr|DeviceManager|FileManager|TrapCalls)|ac(?:512KE|AndPad|C(?:entris6(?:10|50|60AV)|lassic|olorClassic)|II(?:c(?:i|x)|fx|si|v(?:i|m|x)|x)?|Kbd|LC(?:475|5(?:20|75|80)|II(?:I)?)?|OS(?:Compatibility(?:Box(?:Attr|HasSerial|Present|less))?|XQD(?:Text)?)|Plus(?:Kbd)?|Quadra(?:6(?:05|10|30|50|60AV)|700|8(?:00|40AV)|9(?:00|50))|SE(?:030)?|TV|XL|hine(?:Icon|Type))|e(?:diaBay|moryMap(?:Attr|Sparse)|nuMgr(?:A(?:quaLayout(?:Bit|Mask)|ttr)|CGImageMenuTitle(?:Bit|Mask)|M(?:oreThanFiveMenusDeep(?:Bit|Mask)|ultipleItemsWithCommandID(?:Bit|Mask))|Present(?:Bit|Mask)?|RetainsIconRef(?:Bit|Mask)|SendsMenuBoundsToDefProc(?:Bit|Mask))|ssageMgrVersion)|i(?:scAttr|xedMode(?:Attr|CFM68K(?:Has(?:State|Trap))?|PowerPC|Version))|u(?:lti(?:Channels|pleUsersState)|stUseFCBAccessors))|N(?:a(?:meRegistryVersion|tive(?:CPU(?:family|type)|ProcessMgrBit|T(?:imeMgr|ype1FontSupport)))|ew(?:HandleReturnsZeroedMemoryBit|PtrReturnsZeroedMemoryBit)|o(?:FPU|MMU|tification(?:MgrAttr|Present))|uBus(?:Connectors|Present|SlotCount))|O(?:CE(?:SFServerAvailable|T(?:B(?:Available|NativeGlueAvailable|Present)?|oolbox(?:Attr|Version)))|FA2available|S(?:Attr|L(?:CompliantFinder|InSystem)|Table|XFBCCurrentVersion)|pen(?:FirmwareInfo|Tpt(?:A(?:RAPPresent|ppleTalk(?:Loaded(?:Bit|Mask)|Present(?:Bit|Mask)))|IPXSPX(?:Loaded(?:Bit|Mask)|Present(?:Bit|Mask))|Loaded(?:Bit|Mask)|NetworkSetup(?:Legacy(?:Export|Import)|SupportsMultihoming|Version)?|P(?:PPPresent|resent(?:Bit|Mask))|RemoteAccess(?:ClientOnly|Loaded|MPServer|P(?:Server|resent)|Version)?|TCP(?:Loaded(?:Bit|Mask)|Present(?:Bit|Mask))|Versions)?)|riginal(?:ATSUVersion|QD(?:Text)?)|utlineFonts)|P(?:C(?:Card(?:FamilyPresent|HasPowerControl|SupportsCardBus)?|X(?:Attr|Has(?:8and16BitFAT|ProDOS)|NewUI|UseICMapping))|Mgr(?:CPUIdle|DispatchExists|Exists|S(?:CC|ound|upportsAVPowerStateAtSleepWake))|PC(?:DragLibPresent|QuickTimeLibPresent|Supports(?:Incoming(?:AppleTalk|TCP_IP)?|Out(?:Going|going(?:AppleTalk|TCP_IP))|RealTime|TCP_IP)|Toolbox(?:Attr|Present))|S2Keyboard|ar(?:ity(?:Attr|Enabled)|tialRsrcs)|erforma(?:250|4(?:50|6x|7x)|5(?:300|50|80)|6(?:00|3(?:00|60)|400))|hysicalRAMSize(?:InMegabytes)?|layAndRecord|o(?:pup(?:Attr|Present)|rt(?:ADisabled|BDisabled|able(?:2001(?:ANSIKbd|ISOKbd|JISKbd)|SlotPresent|USB(?:ANSIKbd|ISOKbd|JISKbd))?)|wer(?:Book(?:1(?:00|4(?:0(?:0)?|5)|50|6(?:0|5(?:c)?)|70|80(?:c)?|90)|2400|3400|5(?:00PPCUpgrade|20(?:c)?|300|40(?:c)?)|Duo2(?:10|30(?:0)?|50|70c|80(?:c)?)|G3(?:Series(?:2)?)?)|M(?:ac(?:4400(?:_160)?|5(?:2(?:00|60)|400|500)|6(?:100_6(?:0|6)|200|400|500)|7(?:100_(?:66|80)|200|300|500|600)|8(?:100_(?:1(?:00|10|20)|80)|500|600)|9(?:500|600)|Centris6(?:10|50)|G3|LC(?:475|575|630)|NewWorld|Performa(?:47x|57x|63x)|Quadra(?:6(?:10|30|50)|700|800|9(?:00|50)))|gr(?:Attr|Vers))|PC(?:A(?:SArchitecture|ware)|Has(?:64BitSupport|D(?:CB(?:AInstruction|TStreams)|ataStreams)|GraphicsInstructions|S(?:TFIWXInstruction|quareRootInstructions)|VectorInstructions)|IgnoresDCBST|ProcessorFeatures)?))|r(?:o(?:F16(?:ANSIKbd|ISOKbd|JISKbd)|c(?:ClkSpeed(?:MHz)?|essor(?:CacheLineSize|Type)))|tbl(?:ADBKbd|ISOKbd))|wrB(?:k(?:99JISKbd|E(?:K(?:DomKbd|ISOKbd|JISKbd)|xt(?:ADBKbd|ISOKbd|JISKbd))|Sub(?:DomKbd|ISOKbd|JISKbd))|ook(?:ADBKbd|ISOADBKbd)))|Q(?:D(?:3D(?:Present|V(?:ersion|iewer(?:Present)?))?|HasLongRowBytes|Text(?:Features|Version))|TVR(?:C(?:ubicPanosPresent|ylinderPanosPresent)|Mgr(?:Attr|Present|Vers)|ObjMoviesPresent)|u(?:adra(?:6(?:05|10|30|50|60AV)|700|8(?:00|40AV)|9(?:00|50))|ick(?:Time(?:Conferencing(?:Info)?|Features|Streaming(?:Features|Version)|ThreadSafe(?:FeaturesAttr|Graphics(?:Export|Import)|ICM|Movie(?:Export|Import|Playback|Toolbox))|Version)?|draw(?:Features|Version))))|R(?:BVAddr|M(?:F(?:akeAppleMenuItemsRolledIn|orceSysHeapRolledIn)|SupportsFSCalls|TypeIndexOrderingReverse)|OM(?:Size|Version)|e(?:al(?:TempMemory|timeMgr(?:Attr|Present))|sourceMgr(?:Attr|BugFixesAttrs)|visedTimeMgr))|S(?:C(?:C(?:ReadAddr|WriteAddr)|SI(?:PollSIH|SlotBoot)?)|DP(?:FindVersion|PromptVersion|StandardDirectoryVersion)|E(?:30SlotPresent|SlotPresent)|FServer|MP(?:MailerVersion|SPSendLetterVersion)|a(?:feOFAttr|nityCheckResourceFiles)|bitFontSupport|cr(?:apMgr(?:Attr|TranslationAware)|eenCapture(?:Dir|Main)|ipt(?:Count|MgrVersion|ingSupport)|ollingThrottle)|e(?:rialA(?:rbitrationExists|ttr)|tDragImageUpdates)|h(?:eetsAreWindowModal(?:Bit|Mask)|utdown(?:Attributes|HassdOnBootVolUnmount))|lot(?:Attr|MgrExists)|ndPlayDoubleBuffer|o(?:ftwareVendor(?:Apple|Code|Licensee)|und(?:Attr|IOMgrPresent))|p(?:e(?:cificMatchSupport|ech(?:Attr|HasPPCGlue|MgrPresent|Recognition(?:Attr|Version)))|litOS(?:A(?:ttr|ware)|BootDriveIsNetworkVolume|EnablerVolumeIsDifferentFromBootVolume|MachineNameS(?:etToNetworkNameTemp|tartupDiskIsNonPersistent)))|quareMenuBar|t(?:andard(?:File(?:58|Attr|Has(?:ColorIcons|DynamicVolumeAllocation)|TranslationAware|UseGenericIcons)|TimeMgr)|d(?:ADBKbd|ISOADBKbd|NBP(?:Attr|Present|SupportsAutoPosition))|ereo(?:Capability|Input|Mixing))|upports(?:ApplicationURL|FSpResourceFileAlreadyOpenBit|Mirroring)|ys(?:Architecture|DebuggerSupport|ZoneGrowable|temUpdateVersion))|T(?:E(?:1|2|3|4|5|6|Attr|Has(?:GetHiliteRgn|WhiteBackground)|Supports(?:InlineInput|TextObjects))|SM(?:DisplayMgrAwareBit|TE(?:1(?:5(?:2)?)?|Attr|Present|Version)?|doesTSMTEBit|gr(?:15|2(?:0|2|3)|Attr|Version))|VAttr|e(?:le(?:Mgr(?:A(?:ttr|utoAnswer)|IndHandset|NewTELNewSupport|P(?:owerPCSupport|resent)|S(?:ilenceDetect|oundStreams))|phoneSpeechRecognition)|mpMem(?:Support|Tracked)|rmMgr(?:Attr|ErrorString|Present)|xtEditVersion)|h(?:irdParty(?:ANSIKbd|ISOKbd|JISKbd)|read(?:Mgr(?:Attr|Present)|sLibraryPresent))|imeMgrVersion|oolboxTable|ranslation(?:Attr|GetPathAPIAvail|Mgr(?:Exists|HintOrder)|PPCAvail))|U(?:DFSupport|SB(?:A(?:ndy(?:ANSIKbd|ISOKbd|JISKbd)|ttr)|Cosmo(?:ANSIKbd|ISOKbd|JISKbd)|HasIsoch|Pr(?:esent|interSharing(?:Attr(?:Booted|Mask|Running)?|Version(?:Mask)?)|oF16(?:ANSIKbd|ISOKbd|JISKbd))|Version)|n(?:defSelectorErr|known(?:Err|ThirdPartyKbd))|serVisibleMachineName)|V(?:IA(?:1Addr|2Addr)|M(?:Attr|BackingStoreFileRefNum|FilemappingOn|Has(?:LockMemoryForOutput|PagingControl)|Info(?:NoneType|Si(?:mpleType|ze(?:StorageType|Type))|Type)|Present|ZerosPagesBit)|alueImplementedVers|ersion)|W(?:SII(?:CanPrintWithoutPrGeneralBit|Support)|indow(?:LiveResize(?:Bit|Mask)|M(?:gr(?:Attr|Present(?:Bit|Mask)?)|inimizeToDock(?:Bit|Mask)))|orldScriptII(?:Attr|Version))|X86(?:AdditionalFeatures|Features|Has(?:APIC|C(?:ID|LFSH|MOV|X(?:16|8))|D(?:E|S(?:CPL)?)|EST|F(?:PU|XSR)|HTT|M(?:C(?:A|E)|MX|ONITOR|SR|TRR)|P(?:A(?:E|T)|GE|S(?:E(?:36)?|N))|S(?:EP|MX|S(?:E(?:2|3)?)?|upplementalSSE3)|T(?:M(?:2)?|SC)|VM(?:E|X)|xTPR)|ResACPI|Serviced20)))|fpErr|ra(?:bTimeComplete|veUpr(?:E|I|O|U))|uestNotAllowedErr)|h(?:AxisOnly|Menu(?:Cmd|FindErr)|a(?:chek|ndlerNotFoundErr|rdwareConfigErr)|i(?:Archive(?:EncodingCompleteErr|HIObjectIgnoresArchivingErr|KeyNotAvailableErr|TypeMismatchErr)|Object(?:C(?:annotSubclassSingletonErr|lass(?:ExistsErr|Has(?:InstancesErr|SubclassesErr)|IsAbstractErr))|Delegate(?:AlreadyExistsErr|NotFoundErr))|erMenu|ghLevelEventMask|tDev)|m(?:BalloonAborted|CloseViewActive|Help(?:Disabled|ManagerNotInited)|NoBalloonUp|OperationUnsupported|S(?:ameAsLastBalloon|kippedBalloon)|UnknownHelpType|WrongVersion)|our(?:Field|Mask)|r(?:HTMLRenderingLibNotInstalledErr|LeadingZ|MiscellaneousExceptionErr|U(?:RLNotHandledErr|nableToResizeHandleErr))|wParamErr)|i(?:IOAbort(?:Err)?|MemFullErr|c(?:Config(?:InappropriateErr|NotFoundErr)|InternalErr|No(?:MoreWritersErr|Perm|URLErr|thingToOverrideErr)|P(?:ermErr|r(?:ef(?:DataErr|NotFoundErr)|ofileNotFoundErr))|Read(?:OnlyPerm|WritePerm)|T(?:ooManyProfilesErr|runcatedErr)|onItem)|llegal(?:C(?:hannelOSErr|ontrollerOSErr)|InstrumentOSErr|Knob(?:OSErr|ValueOSErr)|NoteChannelOSErr|PartOSErr|ScrapFlavor(?:FlagsErr|SizeErr|TypeErr)|VoiceAllocationOSErr)|n(?:Co(?:llapseBox|ntent)|D(?:esk|rag)|G(?:oAway|row)|MenuBar|NoWindow|ProxyIcon|S(?:tructure|ysWindow)|ToolbarButton|Zoom(?:In|Out)|compatibleVoice|it(?:Dev|IWMErr)|putOutOfBounds|sufficientStackErr|t(?:Arabic|DrawHook|E(?:OLHook|uropean)|HitTestHook|InlineInputTSMTEP(?:ostUpdateHook|reUpdateHook)|Japanese|NWidthHook|OutputMask|Roman|TextWidthHook|W(?:estern|idthHook)|er(?:nal(?:ComponentErr|QuickTimeError|ScrapErr)|ruptsMaskedErr)|lCurrency)|valid(?:Atom(?:ContainerErr|Err|TypeErr)|C(?:hunk(?:Cache|Num)|omponentID)|D(?:ataRef(?:Container)?|uration)|EditState|FolderTypeErr|H(?:andler|otSpotIDErr)|I(?:conRefErr|mageIndexErr|ndexErr)|M(?:edia|ovie)|Node(?:FormatErr|IDErr)|PickerType|Rect|S(?:ample(?:Desc(?:Index|ription)|Num|Table)|prite(?:I(?:DErr|ndexErr)|PropertyErr|WorldPropertyErr))|T(?:ime|ra(?:ck|nslationPathErr))|ViewStateErr))|o(?:Dir(?:Flg|Mask)|Err|QType)|t(?:emDisable|lc(?:D(?:isableKeyScriptSync(?:Mask)?|ualCaret)|S(?:howIcon|ysDirection)))|u(?:Current(?:CurLang|DefLang|Script)|NumberPartsTable|S(?:cript(?:CurLang|DefLang)|ystem(?:CurLang|DefLang|Script))|UnTokenTable|W(?:hiteSpaceList|ord(?:SelectTable|WrapTable))))|k(?:1(?:6(?:B(?:E5(?:55PixelFormat|65PixelFormat)|itCardErr)|LE5(?:55(?:1PixelFormat|PixelFormat)|65PixelFormat))|IndexedGrayPixelFormat|MonochromePixelFormat)|2(?:4(?:BGRPixelFormat|RGBPixelFormat)|Indexed(?:GrayPixelFormat|PixelFormat)|vuyPixelFormat)|3(?:2(?:A(?:BGRPixelFormat|RGBPixelFormat)|B(?:GRAPixelFormat|itHeap)|RGBAPixelFormat)|DMixer(?:AttenuationCurve_(?:Exponential|Inverse|Linear|Power)|Param_(?:Azimuth|Distance|Elevation|Gain|P(?:laybackRate|ost(?:AveragePower|PeakHoldLevel)|re(?:AveragePower|PeakHoldLevel)))|RenderingFlags_(?:ConstantReverbBlend|D(?:istance(?:Attenuation|Diffusion|Filter)|opplerShift)|InterAuralDelay|LinearDistanceAttenuation)))|4Indexed(?:GrayPixelFormat|PixelFormat)|68kInterruptLevelMask|8Indexed(?:GrayPixelFormat|PixelFormat)|A(?:E(?:A(?:ND|bout|ctivate|fter|l(?:iasSelection|l(?:Caps)?|waysInteract)|n(?:swer|y)|pp(?:earanceChanged|lication(?:Class|Died))|rrow(?:At(?:End|Start)|BothEnds)|sk|utoDown)|B(?:e(?:fore|gin(?:Transaction|ning|sWith))|old)|C(?:a(?:n(?:Interact|SwitchLayer)|se(?:ConsiderMask|IgnoreMask|SensEquals)?)|entered|hangeView|l(?:eanUp|o(?:ne|se))|o(?:mmandClass|n(?:densed|tains)|py|reSuite|untElements)|reate(?:Element|Publisher)|ut)|D(?:ata(?:Array|baseSuite)|e(?:activate|bug(?:POSTHeader|ReplyHeader|XML(?:DebugAll|Re(?:quest|sponse)))|faultTimeout|lete|sc(?:Array|ListFactor(?:None|Type(?:AndSize)?)))|i(?:acritic(?:ConsiderMask|IgnoreMask)?|rectCall|skEvent)|o(?:Not(?:AutomaticallyAddAnnotationsToEvent|IgnoreHandler|PromptForUserConsent)|ObjectsExist|Script|nt(?:DisposeOnResume|Execute|Reco(?:nnect|rd))|wn)|rag|uplicateSelection)|E(?:ditGraphic|ject|mpty(?:Trash)?|nd(?:Transaction|sWith)?|quals|rase|xpan(?:ded|sion(?:ConsiderMask|IgnoreMask)?))|F(?:a(?:lse|st)|etchURL|i(?:nder(?:Events|Suite)|rst)|ormulaProtect|ullyJustified)|G(?:e(?:stalt|t(?:ClassInfo|Data(?:Size)?|EventInfo|InfoSelection|PrivilegeSelection|SuiteInfo|URL))|r(?:eaterThan(?:Equals)?|ow))|H(?:TTPProxy(?:HostAttr|PortAttr)|andle(?:Array|SimpleRanges)|i(?:Quality|dden|gh(?:Level|Priority))|yphens(?:ConsiderMask|IgnoreMask)?)|I(?:Do(?:M(?:arking|inimum)|Whose)|S(?:Action(?:Path)?|C(?:lient(?:Address|IP)|ontentType)|F(?:romUser|ullRequest)|GetURL|HTTPSearchArgs|Method|P(?:assword|ostArgs)|Referrer|S(?:criptName|erver(?:Name|Port))|User(?:Agent|Name)|WebStarSuite)|gnore(?:App(?:EventHandler|PhacHandler)|Sys(?:EventHandler|PhacHandler))|mageGraphic|n(?:fo|goreBuiltInEventHandler|ter(?:actWith(?:All|Local|Self)|ceptOpen|netSuite))|sUniform|talic)|K(?:ataHiragana|ey(?:Class|D(?:escArray|own)))|L(?:ast|e(?:ftJustified|ssThan(?:Equals)?)|o(?:calProcess|gOut|wercase))|M(?:a(?:in|keObjectsVisible)|enu(?:Class|Select)|i(?:ddle|scStandards)|o(?:difiable|use(?:Class|Down(?:InBack)?)|ve(?:d)?))|N(?:OT|avigationKey|e(?:verInteract|xt)|o(?:Arrow|Dispatch|Reply|nmodifiable|rmalPriority|tify(?:Recording|St(?:artRecording|opRecording)))?|ullEvent)|O(?:R|SAXSizeResource|pen(?:Application|Contents|Documents|Selection)?|utline)|P(?:a(?:ckedArray|geSetup|s(?:sSubDescs|te))|lain|r(?:evious|int(?:Documents|Selection|Window)?|o(?:cessNonReplyEvents|mise))|u(?:nctuation(?:ConsiderMask|IgnoreMask)?|tAway(?:Selection)?))|Q(?:D(?:Ad(?:M(?:ax|in)|d(?:Over|Pin))|B(?:ic|lend)|Copy|Not(?:Bic|Copy|Or|Xor)|Or|Su(?:b(?:Over|Pin)|pplementalSuite)|Xor)|u(?:eueReply|i(?:ckdrawSuite|t(?:A(?:ll|pplication)|PreserveState|Reason))))|R(?:PCClass|awKey|e(?:allyLogOut|buildDesktopDB|do|gular|moteProcess|openApplication|place|quiredSuite|s(?:ized|olveNestedLists|tart|ume)|ve(?:alSelection|rt))|ightJustified)|S(?:OAPScheme|a(?:meProcess|ve)|cr(?:apEvent|iptingSizeResource)|e(?:lect|t(?:Data|Position))|h(?:a(?:dow|r(?:edScriptHandler|ing))|ow(?:Clipboard|Preferences|RestartDialog|ShutdownDialog)|utDown)|leep|mall(?:Caps|Kana|SystemFontChanged)|o(?:cks(?:4Protocol|5Protocol|HostAttr|P(?:asswordAttr|ortAttr|roxyAttr)|UserAttr)|rt)|pe(?:cialClassProperties|ech(?:D(?:etected|one)|Suite))|t(?:artRecording|op(?:Recording|pedMoving)|rikethrough)|u(?:bscript|perscript|spend)|y(?:nc|stemFontChanged))|T(?:ableSuite|e(?:rminologyExtension|xtSuite)|hemeSwitch|r(?:ansactionTerminated|ue))|U(?:T(?:Apostrophe|ChangesState|DirectParamIsReference|Enum(?:ListIsExclusive|erated|sAreTypes)|Feminine|HasReturningParam|Masculine|NotDirectParamIsTarget|Optional|P(?:aramIs(?:Reference|Target)|lural|ropertyIsReference)|Re(?:adWrite|plyIsReference)|TightBindingFunction|listOfItems)|n(?:d(?:erline|o)|knownSource)|p(?:date)?|se(?:HTTPProxyAttr|RelativeIterators|S(?:ocksAttr|tandardDispatch)|rTerminology))|Vi(?:ewsFontChanged|rtualKey)|W(?:a(?:itReply|keUpEvent|ntReceipt)|h(?:iteSpace(?:ConsiderMask|IgnoreMask)?|oleWordEquals)|indowClass)|XMLRPCScheme|Yes|Z(?:enkakuHankaku|oom(?:In|Out)?))|FP(?:ExtendedFlagsAlternateAddressMask|ServerIcon|Tag(?:Length(?:DDP|IP(?:Port)?)|Type(?:D(?:DP|NS)|IP(?:Port)?)))|H(?:Intern(?:alErr|etConfigPrefErr)|TOCType(?:Developer|User))|LM(?:D(?:eferSwitchErr|uplicateModuleErr)|GroupNotFoundErr|In(?:stallationErr|ternalErr)|Location(?:NotFoundErr|sFolderType)|Module(?:CommunicationErr|sFolderType)|NoSuchModuleErr|PreferencesFolderType|RebootFlagsLevelErr)|NKRCurrentVersion|RM(?:M(?:ountVol|ultVols)|NoUI|Search(?:More|RelFirst)?|TryFileIDFirst)|S(?:A(?:dd|nd|ppleScriptSuite)|C(?:o(?:m(?:es(?:After|Before)|ment(?:Event)?)|n(?:catenate|siderReplies(?:ConsiderMask|IgnoreMask)?|tains))|urrentApplication)|D(?:efault(?:M(?:ax(?:HeapSize|StackSize)|in(?:HeapSize|StackSize))|Preferred(?:HeapSize|StackSize))|ivide)|E(?:ndsWith|qual|rrorEventCode|xcluding)|GreaterThan(?:OrEqual)?|HasOpenHandler|I(?:mporting|nitializeEventCode)|L(?:aunchEvent|essThan(?:OrEqual)?)|M(?:agic(?:EndTellEvent|TellEvent)|inimumVersion|ultiply)|N(?:egate|ot(?:Equal)?|um(?:berOfSourceStyles|ericStrings(?:ConsiderMask|IgnoreMask)?))|Or|P(?:ower|repositionalSubroutine)|Quotient|Remainder|S(?:criptEditorSuite|elect(?:CopySourceAttributes|Get(?:AppTerminology(?:Obsolete)?|Handler(?:Names|Obsolete)?|Property(?:Names|Obsolete)?|S(?:ourceStyle(?:Names|s)|ysTerminology))|Init|Set(?:Handler(?:Obsolete)?|Property(?:Obsolete)?|Source(?:Attributes|Styles)))|ourceStyle(?:ApplicationKeyword|C(?:lass|omment)|Dynamic(?:Class|E(?:numValue|ventName)|P(?:arameterName|roperty))|E(?:numValue|ventName)|L(?:anguageKeyword|iteral)|NormalText|ObjectSpecifier|P(?:arameterName|roperty)|String|U(?:ncompiledText|serSymbol))|t(?:art(?:LogEvent|sWith)|opLogEvent)|ub(?:routineEvent|tract))|TypeNamesSuite|UseEventCode)|TS(?:BoldQDStretch|CubicCurveType|DeletedGlyphcode|F(?:ileReferenceFilterSelector|lat(?:DataUstl(?:CurrentVersion|Version(?:0|1|2))|tenedFontSpecifierRawNameData)|ont(?:AutoActivation(?:Ask|D(?:efault|isabled)|Enabled)|Cont(?:ainerRefUnspecified|ext(?:Global|Local|Unspecified))|F(?:amilyRefUnspecified|ilter(?:CurrentVersion|Selector(?:Font(?:ApplierFunction|Family(?:ApplierFunction)?)|Generation|Unspecified))|ormatUnspecified)|Notify(?:Action(?:DirectoriesChanged|FontsChanged)|Option(?:Default|ReceiveWhileSuspended))|RefUnspecified))|G(?:enerationUnspecified|lyphInfo(?:AppleReserved|ByteSizeMask|HasImposedWidth|Is(?:Attachment|LTHanger|RBHanger|WhiteSpace)|TerminatorGlyph))|I(?:nvalid(?:Font(?:Access|ContainerAccess|FamilyAccess|TableAccess)|GlyphAccess)|t(?:alicQDSkew|eration(?:Completed|ScopeModified)))|Line(?:Appl(?:eReserved|yAntiAliasing)|BreakToNearestCharacter|Disable(?:A(?:ll(?:BaselineAdjustments|GlyphMorphing|Justification|KerningAdjustments|LayoutOperations|TrackingAdjustments)|utoAdjustDisplayPos)|NegativeJustification)|F(?:illOutToWidth|ractDisable)|HasNo(?:Hangers|OpticalAlignment)|I(?:gnoreFontLeading|mposeNoAngleForEnds|sDisplayOnly)|KeepSpacesOutOfMargin|LastNoJustification|No(?:AntiAliasing|LayoutOptions|SpecialJustification)|TabAdjustEnabled|Use(?:DeviceMetrics|QDRendering))|NoTracking|O(?:ptionFlags(?:ActivateDisabled|ComposeFontPostScriptName|D(?:efault(?:Scope)?|oNotNotify)|I(?:ncludeDisabledMask|terat(?:eByPrecedenceMask|ionScopeMask))|ProcessSubdirectories|Re(?:cordPersistently|strictedScope)|U(?:nRestrictedScope|se(?:DataFork(?:AsResourceFork)?|ResourceFork)))|therCurveType)|Qu(?:adCurveType|eryActivateFontMessage)|RadiansFactor|Style(?:Appl(?:eReserved|y(?:AntiAliasing|Hints))|No(?:AntiAliasing|Hinting|Options))|U(?:A(?:fterWithStreamShiftTag|scentTag)|B(?:a(?:ckgroundC(?:allback|olor)|dStreamErr|selineClassTag)|eforeWithStreamShiftTag|usyObjectErr|y(?:C(?:haracter(?:Cluster)?|luster)|TypographicCluster|Word))|C(?:GContextTag|enterTab|learAll|o(?:lorTag|ordinateOverflowErr)|rossStreamShiftTag)|D(?:ataStreamUnicodeStyledText|e(?:c(?:imalTab|ompositionFactorTag)|faultFontFallbacks|scentTag)|irectData(?:AdvanceDeltaFixedArray|BaselineDeltaFixedArray|DeviceDeltaSInt16Array|LayoutRecordATSLayoutRecord(?:Current|Version1)|Style(?:IndexUInt16Array|SettingATSUStyleSettingRefArray)))|F(?:lattenOptionNoOptionsMask|o(?:nt(?:MatrixTag|Tag|s(?:Matched|NotMatched))|rceHangingTag)|rom(?:FollowingLayout|PreviousLayout|TextBeginning))|GlyphSelectorTag|HangingInhibitFactorTag|I(?:mposeWidthTag|nvalid(?:Attribute(?:SizeErr|TagErr|ValueErr)|Ca(?:cheErr|llInsideCallbackErr)|Font(?:Err|FallbacksErr|ID)|StyleErr|Text(?:LayoutErr|RangeErr)))|KerningInhibitFactorTag|L(?:a(?:ng(?:RegionTag|uageTag)|st(?:Err|ResortOnlyFallback)|youtOperation(?:AppleReserved|BaselineAdjustment|CallbackStatus(?:Continue|Handled)|Justification|KerningAdjustment|Morph|None|OverrideTag|PostLayoutAdjustment|TrackingAdjustment))|e(?:adingTag|ftT(?:ab|oRightBaseDirection))|ine(?:AscentTag|B(?:aselineValuesTag|reakInWord)|D(?:e(?:cimalTabCharacterTag|scentTag)|irectionTag)|F(?:lushFactorTag|ontFallbacksTag)|HighlightCGColorTag|JustificationFactorTag|La(?:ng(?:RegionTag|uageTag)|youtOptionsTag)|RotationTag|T(?:extLocatorTag|runcationTag)|WidthTag)|owLevelErr)|Max(?:ATSUITagValue|LineTag|StyleTag)|N(?:o(?:C(?:aretAngleTag|orrespondingFontErr)|Font(?:CmapAvailableErr|NameErr|ScalerAvailableErr)|LigatureSplitTag|OpticalAlignmentTag|S(?:elector|pecialJustificationTag|tyleRunsAssignedErr)|tSetErr)|umberTabTypes)|OutputBufferTooSmallErr|PriorityJustOverrideTag|Q(?:D(?:BoldfaceTag|CondensedTag|ExtendedTag|ItalicTag|UnderlineTag)|uickDrawTextErr)|R(?:GBAlphaColorTag|ightT(?:ab|oLeftBaseDirection))|S(?:equentialFallbacks(?:Exclusive|Preferred)|izeTag|t(?:rongly(?:Horizontal|Vertical)|yle(?:Contain(?:edBy|s)|D(?:oubleLineCount|ropShadow(?:BlurOptionTag|ColorOptionTag|OffsetOptionTag|Tag))|Equals|RenderingOptionsTag|S(?:ingleLineCount|trikeThrough(?:Co(?:lorOptionTag|untOptionTag)|Tag))|TextLocatorTag|Un(?:derlineCo(?:lorOptionTag|untOptionTag)|equal)))|uppressCrossKerningTag)|T(?:oTextEnd|r(?:ackingTag|unc(?:FeatNoSquishing|ate(?:End|Middle|None|S(?:pecificationMask|tart)))))|U(?:n(?:FlattenOptionNoOptionsMask|supportedStreamFormatErr)|se(?:GrafPortPenLoc|LineControlWidth))|VerticalCharacterTag|se(?:CaretOrigins|DeviceOrigins|FractionalOrigins|GlyphAdvance|LineHeight|OriginFlags)))|U(?:Gr(?:aphErr_(?:CannotDoInCurrentContext|Invalid(?:AudioUnit|Connection)|NodeNotFound|OutputNodeErr)|oupParameterID_(?:All(?:NotesOff|SoundOff)|ChannelPressure|DataEntry(?:_LSB)?|Expression(?:_LSB)?|Foot(?:_LSB)?|KeyPressure(?:_(?:FirstKey|LastKey))?|ModWheel(?:_LSB)?|P(?:an(?:_LSB)?|itchBend)|ResetAllControllers|S(?:ostenuto|ustain)|Volume(?:_LSB)?))|LowShelfParam_(?:CutoffFrequency|Gain)|MIDISynthProperty_EnablePreload|N(?:BandEQ(?:FilterType_(?:2ndOrderButterworth(?:HighPass|LowPass)|Band(?:Pass|Stop)|HighShelf|LowShelf|Parametric|Resonant(?:High(?:Pass|Shelf)|Low(?:Pass|Shelf)))|P(?:aram_(?:B(?:andwidth|ypassBand)|F(?:ilterType|requency)|G(?:ain|lobalGain))|roperty_(?:BiquadCoefficients|MaxNumberOfBands|NumberOfBands)))|et(?:ReceiveP(?:aram_(?:NumParameters|Status)|roperty_(?:Hostname|Password))|S(?:end(?:NumPresetFormats|P(?:aram_(?:NumParameters|Status)|r(?:esetFormat_(?:AAC_(?:128kbpspc|32kbpspc|4(?:0kbpspc|8kbpspc)|64kbpspc|80kbpspc|96kbpspc|LD_(?:32kbpspc|4(?:0kbpspc|8kbpspc)|64kbpspc))|IMA4|Lossless(?:16|24)|PCM(?:Float32|Int(?:16|24))|ULaw)|operty_(?:Disconnect|P(?:assword|ortNum)|ServiceName|TransmissionFormat(?:Index)?))))|tatus_(?:Connect(?:ed|ing)|Listening|NotConnected|Overflow|Underflow)))|odeInteraction_(?:Connection|InputCallback))|Parameter(?:Listener_AnyParameter|MIDIMapping_(?:Any(?:ChannelFlag|NoteFlag)|Bipolar(?:_On)?|SubRange|Toggle))|Sampler(?:P(?:aram_(?:CoarseTuning|FineTuning|Gain|Pan)|roperty_(?:BankAndPreset|Load(?:AudioFiles|Instrument|PresetFromBank)))|_Default(?:BankLSB|MelodicBankMSB|PercussionBankMSB))|VoiceIO(?:Err_UnexpectedNumberOfInputChannels|Property_(?:BypassVoiceProcessing|MuteOutput|VoiceProcessingEnableAGC)))|VL(?:I(?:nOrder|s(?:Le(?:af|ftBranch)|RightBranch|Tree))|NullNode|P(?:ostOrder|reOrder))|X(?:CopyMultipleAttributeOptionStopOnError|Error(?:A(?:PIDisabled|ctionUnsupported|ttributeUnsupported)|CannotComplete|Failure|I(?:llegalArgument|nvalidUIElement(?:Observer)?)|No(?:Value|t(?:EnoughPrecision|Implemented|ification(?:AlreadyRegistered|NotRegistered|Unsupported)))|ParameterizedAttributeUnsupported|Success)|MenuItemModifier(?:Control|No(?:Command|ne)|Option|Shift)|UnderlineStyle(?:Double|None|Single|Thick))|bbrevSquaredLigaturesO(?:ffSelector|nSelector)|c(?:c(?:essException|ountKCItemAttr)|tivateAnd(?:HandleClick|IgnoreClick))|dd(?:KCEvent(?:Mask)?|ressKCItemAttr)|l(?:ert(?:Caution(?:Alert|BadgeIcon|Icon)|Default(?:CancelText|O(?:KText|therText))|Flags(?:AlertIsMovable|Use(?:Co(?:mpositing|ntrolHierarchy)|Theme(?:Background|Controls)))|Note(?:Alert|Icon)|PlainAlert|St(?:dAlert(?:CancelButton|HelpButton|O(?:KButton|therButton))|op(?:Alert|Icon))|VariantCode|WindowClass)|i(?:asBadgeIcon|gn(?:AbsoluteCenter|Bottom(?:Left|Right)?|Center(?:Bottom|Left|Right|Top)|HorizontalCenter|Left|None|Right|Top(?:Left|Right)?|VerticalCenter))|l(?:CapsSelector|LowerCaseSelector|PPDDomains|Typ(?:eFeaturesO(?:ffSelector|nSelector)|ographicFeaturesType)|WindowClasses)|readySavedStateErr|t(?:HalfWidthTextSelector|P(?:lainWindowClass|roportionalTextSelector)|ernate(?:HorizKanaO(?:ffSelector|nSelector)|KanaType|VertKanaO(?:ffSelector|nSelector)))|ways(?:Authenticate|SendSubject))|n(?:dConnections|notationType|y(?:AuthType|Component(?:FlagsMask|Manufacturer|SubType|Type)|P(?:ort|rotocol)|TransactionID))|pp(?:PackageAliasType|earance(?:EventClass|Folder(?:Icon|Type)|Part(?:DownButton|Indicator|LeftButton|Meta(?:Disabled|Inactive|None)|Page(?:DownArea|LeftArea|RightArea|UpArea)|RightButton|UpButton)|Region(?:C(?:loseBox|o(?:llapseBox|ntent))|Drag|Grow|Structure|T(?:itle(?:Bar|ProxyIcon|Text)|oolbarButton)|ZoomBox))|l(?:e(?:ExtrasFolder(?:Icon|Type)|JapaneseDictionarySignature|Lo(?:go(?:CharCode|Icon|Unicode)|sslessFormatFlag_(?:16BitSourceData|2(?:0BitSourceData|4BitSourceData)|32BitSourceData))|M(?:anufacturer|enu(?:Folder(?:AliasType|Icon(?:Resource)?|Type)|Icon))|S(?:cript(?:BadgeIcon|Subtype)|hare(?:AuthenticationFolderType|PasswordKCItemClass|SupportFolderType))|Talk(?:Icon|ZoneIcon)|shareAutomountServerAliasesFolderType)|ication(?:AliasType|CPAliasType|DAAliasType|SupportFolder(?:Icon|Type)|ThreadID|WindowKind|sFolder(?:Icon|Type))))|s(?:sistantsFolder(?:Icon|Type)|teriskToMultiplyO(?:ffSelector|nSelector)|ync(?:Eject(?:Complete|InProgress)|Mount(?:Complete|InProgress)|Unmount(?:Complete|InProgress)))|t(?:SpecifiedOrigin|temptDupCardEntryErr)|u(?:dio(?:A(?:ggregateDevice(?:ClassID|Property(?:ActiveSubDeviceList|C(?:lockDevice|omposition)|FullSubDeviceList|MasterSubDevice))|lertSoundsFolderType)|B(?:alanceFadeType_(?:EqualPower|MaxUnityGain)|o(?:o(?:leanControl(?:ClassID|PropertyValue)|tChimeVolumeControlClassID)|x(?:ClassID|Property(?:Acqui(?:red|sitionFailed)|BoxUID|ClockDeviceList|DeviceList|Has(?:Audio|MIDI|Video)|IsProtected|TransportType))))|C(?:hannel(?:Bit_(?:Center(?:Surround|Top(?:Front|Middle|Rear))?|L(?:FEScreen|eft(?:Center|Surround(?:Direct)?|Top(?:Front|Middle|Rear))?)|Right(?:Center|Surround(?:Direct)?|Top(?:Front|Middle|Rear))?|Top(?:Back(?:Center|Left|Right)|CenterSurround)|VerticalHeight(?:Center|Left|Right))|Coordinates_(?:Azimuth|BackFront|D(?:istance|ownUp)|Elevation|LeftRight)|Flags_(?:AllOff|Meters|RectangularCoordinates|SphericalCoordinates)|La(?:bel_(?:Ambisonic_(?:W|X|Y|Z)|B(?:eginReserved|inaural(?:Left|Right))|C(?:enter(?:Surround(?:Direct)?|Top(?:Front|Middle|Rear))?|lickTrack)|Di(?:alogCentricMix|screte(?:_(?:0|1(?:0|1|2|3|4|5)?|2|3|4|5|6(?:5535)?|7|8|9))?)|EndReserved|ForeignLanguage|H(?:OA_ACN(?:_(?:0|1(?:0|1|2|3|4|5)?|2|3|4|5|6(?:5024)?|7|8|9))?|aptic|ea(?:dphones(?:Left|Right)|ringImpaired))|L(?:FE(?:2|Screen)|eft(?:Center|Surround(?:Direct)?|To(?:p(?:Front|Middle|Rear)|tal)|Wide)?)|M(?:S_(?:Mid|Side)|ono)|Narration|R(?:earSurround(?:Left|Right)|ight(?:Center|Surround(?:Direct)?|To(?:p(?:Front|Middle|Rear)|tal)|Wide)?)|Top(?:Back(?:Center|Left|Right)|CenterSurround)|U(?:n(?:known|used)|seCoordinates)|VerticalHeight(?:Center|Left|Right)|XY_(?:X|Y))|youtTag_(?:A(?:AC_(?:3_0|4_0|5_(?:0|1)|6_(?:0|1)|7_(?:0|1(?:_(?:B|C))?)|Octagonal|Quadraphonic)|C3_(?:1_0_1|2_1_1|3_(?:0(?:_1)?|1(?:_1)?))|mbisonic_B_Format|tmos_(?:5_1_2|7_1_4|9_1_6)|udioUnit_(?:4|5(?:_(?:0|1))?|6(?:_(?:0|1))?|7_(?:0(?:_Front)?|1(?:_Front)?)|8))|B(?:eginReserved|inaural)|Cube|D(?:TS_(?:3_1|4_1|6_(?:0_(?:A|B|C)|1_(?:A|B|C|D))|7_(?:0|1)|8_(?:0_(?:A|B)|1_(?:A|B)))|VD_(?:0|1(?:0|1|2|3|4|5|6|7|8|9)?|2(?:0)?|3|4|5|6|7|8|9)|iscreteInOrder)|E(?:AC(?:3_(?:6_1_(?:A|B|C)|7_1_(?:A|B|C|D|E|F|G|H))|_(?:6_0_A|7_0_A))|magic_Default_7_1|ndReserved)|H(?:OA_ACN_(?:N3D|SN3D)|exagonal)|ITU_(?:1_0|2_(?:0|1|2)|3_(?:0|1|2(?:_1)?|4_1))|M(?:PEG_(?:1_0|2_0|3_0_(?:A|B)|4_0_(?:A|B)|5_(?:0_(?:A|B|C|D)|1_(?:A|B|C|D))|6_1_A|7_1_(?:A|B|C))|atrixStereo|idSide|ono)|Octagonal|Pentagonal|Quadraphonic|S(?:MPTE_DTV|tereo(?:Headphones)?)|TMH_10_2_(?:full|std)|U(?:nknown|seChannel(?:Bitmap|Descriptions))|WAVE_(?:2_1|3_0|4_0_(?:A|B)|5_(?:0_(?:A|B)|1_(?:A|B))|6_1|7_1)|XY)))|l(?:ipLightControlClassID|ock(?:Device(?:ClassID|Property(?:AvailableNominalSampleRates|C(?:lockDomain|ontrolList)|Device(?:Is(?:Alive|Running)|UID)|Latency|NominalSampleRate|TransportType))|Source(?:Control(?:ClassID|PropertyItemKind)|ItemKindInternal)))|o(?:dec(?:AppendInput(?:BufferListSelect|DataSelect)|B(?:ad(?:DataError|PropertySizeError)|itRate(?:ControlMode_(?:Constant|LongTermAverage|Variable(?:Constrained)?)|Format(?:_(?:ABR|CBR|VBR))?))|D(?:elayMode_(?:Compatibility|Minimum|Optimal)|oesSampleRateConversion)|ExtendFrequencies|GetProperty(?:InfoSelect|Select)|I(?:llegalOperationError|n(?:itializeSelect|putFormatsForOutputFormat))|No(?:Error|tEnoughBufferSpaceError)|Output(?:FormatsForInputFormat|Precedence(?:BitRate|None|SampleRate)?)|Pr(?:imeMethod_(?:No(?:ne|rmal)|Pre)|o(?:duceOutput(?:BufferListSelect|DataSelect|Packet(?:AtEOF|Failure|NeedsMoreInputData|Success(?:HasMore)?))|perty(?:A(?:djustLocalQuality|pplicable(?:BitRateRange|InputSampleRates|OutputSampleRates)|vailable(?:BitRate(?:Range|s)|Input(?:ChannelLayout(?:Tags|s)|SampleRates)|NumberChannels|Output(?:ChannelLayout(?:Tags|s)|SampleRates)))|BitRate(?:ControlMode|ForVBR)|Current(?:Input(?:ChannelLayout|Format|SampleRate)|Output(?:ChannelLayout|Format|SampleRate)|TargetBitRate)|D(?:elayMode|oesSampleRateConversion|ynamicRangeControlMode)|EmploysDependentPackets|Format(?:CFString|Info|List)|HasVariablePacketByteSizes|I(?:nput(?:BufferSize|ChannelLayout|FormatsForOutputFormat)|sInitialized)|M(?:a(?:gicCookie|nufacturerCFString|ximumPacketByteSize)|inimum(?:DelayMode|Number(?:InputPackets|OutputPackets)))|NameCFString|Output(?:ChannelLayout|FormatsForInputFormat)|P(?:a(?:cket(?:FrameSize|SizeLimitForVBR)|ddedZeros)|r(?:ime(?:Info|Method)|ogramTargetLevel(?:Constant)?))|QualitySetting|Re(?:commendedBitRateRange|quiresPacketDescription)|S(?:ettings|oundQualityForVBR|upported(?:InputFormats|OutputFormats))|UsedInputBufferSize|ZeroFramesPadded)))|Quality_(?:High|Low|M(?:ax|edium|in))|ResetSelect|S(?:etPropertySelect|tateError)|U(?:n(?:initializeSelect|knownPropertyError|s(?:pecifiedError|upportedFormatError))|seRecommendedSampleRate))|mponent(?:Err_Instance(?:Invalidated|TimedOut)|Flag_Unsearchable|ValidationResult_(?:Failed|Passed|TimedOut|Un(?:authorizedError_(?:Init|Open)|known))|sFolderType)|n(?:trol(?:ClassID|Property(?:Element|Scope|Variant))|verter(?:A(?:pplicableEncode(?:BitRates|SampleRates)|vailableEncode(?:BitRates|ChannelLayoutTags|SampleRates))|C(?:hannelMap|o(?:decQuality|mpressionMagicCookie)|urrent(?:InputStreamDescription|OutputStreamDescription))|DecompressionMagicCookie|E(?:ncode(?:AdjustableSampleRate|BitRate)|rr_(?:BadPropertySizeError|FormatNotSupported|In(?:putSampleRateOutOfRange|valid(?:InputSize|OutputSize))|O(?:perationNotSupported|utputSampleRateOutOfRange)|PropertyNotSupported|RequiresPacketDescriptionsError|UnspecifiedError))|InputChannelLayout|OutputChannelLayout|Pr(?:ime(?:Info|Method)|operty(?:BitDepthHint|Calculate(?:InputBufferSize|OutputBufferSize)|Dither(?:BitDepth|ing)|FormatList|InputCodecParameters|M(?:aximum(?:Input(?:BufferSize|PacketSize)|OutputPacketSize)|inimum(?:InputBufferSize|OutputBufferSize))|OutputCodecParameters|Settings))|Quality_(?:High|Low|M(?:ax|edium|in))|SampleRateConverter(?:Algorithm|Complexity(?:_(?:Linear|M(?:astering|inimumPhase)|Normal))?|InitialPhase|Quality)))))|D(?:ata(?:DestinationControlClassID|SourceControlClassID)|e(?:coderComponentType|vice(?:ClassID|P(?:ermissionsError|ro(?:cessorOverload|perty(?:A(?:ctualSampleRate|vailableNominalSampleRates)|Buffer(?:FrameSize(?:Range)?|Size(?:Range)?)|C(?:hannel(?:CategoryName(?:CFString)?|N(?:ame(?:CFString)?|ominalLineLevel(?:NameForID(?:CFString)?|s)?|umberName(?:CFString)?))|l(?:ipLight|ock(?:D(?:evice|omain)|Source(?:KindForID|NameForID(?:CFString)?|s)?))|onfigurationApplication)|D(?:ataSource(?:KindForID|NameForID(?:CFString)?|s)?|evice(?:CanBeDefault(?:Device|SystemDevice)|HasChanged|Is(?:Alive|Running(?:Somewhere)?)|Manufacturer(?:CFString)?|Name(?:CFString)?|UID)|riverShouldOwniSub)|H(?:ighPassFilterSetting(?:NameForID(?:CFString)?|s)?|ogMode)|I(?:O(?:CycleUsage|ProcStreamUsage|StoppedAbnormally)|con|sHidden)|JackIsConnected|L(?:atency|istenback)|M(?:odelUID|ute)|NominalSampleRate|P(?:ha(?:ntomPower|seInvert)|l(?:ayThru(?:Destination(?:NameForID(?:CFString)?|s)?|S(?:olo|tereoPan(?:Channels)?)|Volume(?:Decibels(?:ToScalar(?:TransferFunction)?)?|RangeDecibels|Scalar(?:ToDecibels)?))?|ugIn)|referredChannel(?:Layout|sForStereo))|Re(?:gisterBufferList|latedDevices)|S(?:afetyOffset|cope(?:Input|Output|PlayThrough)|olo|t(?:ereoPan(?:Channels)?|ream(?:Configuration|Format(?:Match|Supported|s)?|s))|u(?:b(?:Mute|Volume(?:Decibels(?:ToScalar(?:TransferFunction)?)?|RangeDecibels|Scalar(?:ToDecibels)?))|pportsMixing))|T(?:alkback|ransportType)|UsesVariableBufferFrameSizes|Volume(?:Decibels(?:ToScalar(?:TransferFunction)?)?|RangeDecibels|Scalar(?:ToDecibels)?))))|StartTime(?:DontConsult(?:DeviceFlag|HALFlag)|IsInputFlag)|TransportType(?:A(?:VB|ggregate|irPlay|utoAggregate)|B(?:luetooth(?:LE)?|uiltIn)|DisplayPort|FireWire|HDMI|PCI|Thunderbolt|U(?:SB|nknown)|Virtual)|Un(?:known|supportedFormatError)))|igidesignFolderType)|En(?:coderComponentType|dPoint(?:ClassID|Device(?:ClassID|Property(?:Composition|EndPointList|IsPrivate))))|F(?:ile(?:3GP(?:2Type|Type)|A(?:AC_ADTSType|C3Type|IF(?:CType|FType)|MRType)|BadPropertySizeError|C(?:AFType|loseSelect|o(?:mponent_(?:Available(?:FormatIDs|StreamDescriptionsForFormat)|Can(?:Read|Write)|ExtensionsForType|F(?:astDispatchTable|ileTypeName)|HFSTypeCodesForType|MIMETypesForType|UTIsForType)|untUserDataSelect)|reate(?:Select|URLSelect))|D(?:ataIsThisFormatSelect|oesNotAllow64BitDataSizeError)|E(?:ndOfFileError|xtensionIsThisFormatSelect)|F(?:LACType|ile(?:DataIsThisFormatSelect|IsThisFormatSelect|NotFoundError)|lags_(?:DontPageAlignAudioData|EraseFile))|G(?:et(?:GlobalInfoS(?:elect|izeSelect)|Property(?:InfoSelect|Select)|UserDataS(?:elect|izeSelect))|lobalInfo_(?:A(?:ll(?:Extensions|HFSTypeCodes|MIMETypes|UTIs)|vailable(?:FormatIDs|StreamDescriptionsForFormat))|ExtensionsForType|FileTypeName|HFSTypeCodesForType|MIMETypesForType|ReadableTypes|TypesFor(?:Extension|HFSTypeCode|MIMEType|UTI)|UTIsForType|WritableTypes))|In(?:itialize(?:Select|WithCallbacksSelect)|valid(?:ChunkError|FileError|Packet(?:DependencyError|OffsetError)))|L(?:ATMInLOASType|oopDirection_(?:Backward|Forward(?:AndBackward)?|NoLooping))|M(?:4(?:AType|BType)|P(?:1Type|2Type|3Type|EG4Type)|arkerType_Generic)|N(?:extType|otOp(?:enError|timizedError))|Op(?:e(?:n(?:Select|URLSelect|WithCallbacksSelect)|rationNotSupportedError)|timizeSelect)|P(?:ermissionsError|ositionError|roperty(?:A(?:lbumArtwork|udio(?:Data(?:ByteCount|PacketCount)|TrackCount))|B(?:itRate|yteToPacket)|Ch(?:annelLayout|unkIDs)|D(?:ata(?:Format(?:Name)?|Offset)|eferSizeUpdates)|EstimatedDuration|F(?:ileFormat|ormatList|rameToPacket)|I(?:D3Tag|nfoDictionary|sOptimized)|Ma(?:gicCookieData|rkerList|ximumPacketSize)|NextIndependentPacket|P(?:acket(?:RangeByteCountUpperBound|SizeUpperBound|T(?:ableInfo|o(?:Byte|DependencyInfo|Frame|RollDistance)))|reviousIndependentPacket)|Re(?:gionList|s(?:erveDuration|trictsRandomAccess))|SourceBitDepth|UseAudioTrack))|R(?:F64Type|e(?:ad(?:BytesSelect|P(?:acket(?:DataSelect|sSelect)|ermission)|WritePermission)|gionFlag_(?:LoopEnable|Play(?:Backward|Forward))|moveUserDataSelect))|S(?:et(?:PropertySelect|UserDataSelect)|oundDesigner2Type|tream(?:Error_(?:BadPropertySize|D(?:ataUnavailable|iscontinuityCantRecover)|I(?:llegalOperation|nvalid(?:File|PacketOffset))|NotOptimized|Uns(?:pecifiedError|upported(?:DataFormat|FileType|Property))|ValueUnknown)|P(?:arseFlag_Discontinuity|roperty(?:Flag_(?:CacheProperty|PropertyIsCached)|_(?:A(?:udioData(?:ByteCount|PacketCount)|verageBytesPerPacket)|B(?:itRate|yteToPacket)|ChannelLayout|Data(?:Format|Offset)|F(?:ileFormat|ormatList|rameToPacket)|InfoDictionary|Ma(?:gicCookieData|ximumPacketSize)|NextIndependentPacket|P(?:acket(?:SizeUpperBound|T(?:ableInfo|o(?:Byte|DependencyInfo|Frame|RollDistance)))|reviousIndependentPacket)|Re(?:adyToProducePackets|strictsRandomAccess))))|SeekFlag_OffsetIsEstimated))|Uns(?:pecifiedError|upported(?:DataFormatError|FileTypeError|PropertyError))|W(?:AVEType|rite(?:BytesSelect|P(?:acketsSelect|ermission))))|ormat(?:60958AC3|A(?:C3|ES3|Law|MR(?:_WB)?|pple(?:IMA4|Lossless)|udible)|Bad(?:PropertySizeError|SpecifierSizeError)|DVIIntelIMA|EnhancedAC3|F(?:LAC|lag(?:Is(?:AlignedHigh|BigEndian|Float|Non(?:Interleaved|Mixable)|Packed|SignedInteger)|s(?:A(?:reAllClear|udioUnitCanonical)|Canonical|Native(?:Endian|FloatPacked))))|LinearPCM|M(?:ACE(?:3|6)|IDIStream|PEG(?:4(?:AAC(?:_(?:ELD(?:_(?:SBR|V2))?|HE(?:_V2)?|LD|Spatial))?|CELP|HVXC|TwinVQ)|D_USAC|Layer(?:1|2|3))|icrosoftGSM)|Opus|P(?:arameterValueStream|roperty_(?:A(?:SBDFrom(?:ESDS|MPEGPacket)|reChannelLayoutsEquivalent|vailableEncode(?:BitRates|ChannelLayoutTags|NumberChannels|SampleRates))|B(?:alanceFade|itmapForLayoutTag)|Channel(?:Layout(?:F(?:or(?:Bitmap|Tag)|romESDS)|Hash|Name|SimpleName)|Map|Name|ShortName)|Decode(?:FormatIDs|rs)|Encode(?:FormatIDs|rs)|F(?:irstPlayableFormatFromList|ormat(?:EmploysDependentPackets|I(?:nfo|s(?:E(?:ncrypted|xternallyFramed)|VBR))|List|Name))|ID3Tag(?:Size|ToDictionary)|MatrixMixMap|NumberOfChannelsForLayout|OutputFormatList|PanningMatrix|Tag(?:ForChannelLayout|sForNumberOfChannels)|ValidateChannelLayout))|Q(?:Design(?:2)?|UALCOMM)|TimeCode|U(?:Law|n(?:knownFormatError|s(?:pecifiedError|upported(?:DataFormatError|PropertyError))))|iLBC))|H(?:ardware(?:Bad(?:DeviceError|ObjectError|PropertySizeError|StreamError)|IllegalOperationError|No(?:Error|tRunningError)|P(?:owerHint(?:FavorSavingPower|None)|roperty(?:Bo(?:otChimeVolume(?:Decibels(?:ToScalar(?:TransferFunction)?)?|RangeDecibels|Scalar(?:ToDecibels)?)|xList)|ClockDeviceList|De(?:fault(?:InputDevice|OutputDevice|SystemOutputDevice)|vice(?:ForUID|s))|HogModeIsAllowed|IsInitingOrExiting|MixStereoToMono|P(?:lugIn(?:ForBundleID|List)|owerHint|rocessIs(?:Audible|Master))|RunLoop|S(?:erviceRestarted|leepingIsAllowed)|Trans(?:late(?:BundleIDTo(?:PlugIn|TransportManager)|UIDTo(?:Box|ClockDevice|Device))|portManagerList)|U(?:nloadingIsAllowed|ser(?:IDChanged|SessionIsActiveOrHeadless))))|Service(?:DeviceProperty_VirtualMaster(?:Balance|Volume)|Property_ServiceRestarted)|Un(?:knownPropertyError|s(?:pecifiedError|upportedOperationError)))|ighPassFilterControlClassID)|ISubOwnerControlClassID|JackControlClassID|L(?:FE(?:MuteControlClassID|VolumeControlClassID)|evelControl(?:ClassID|Property(?:Convert(?:DecibelsToScalar|ScalarToDecibels)|Decibel(?:Range|Value|sToScalarTransferFunction)|ScalarValue)|TranferFunction(?:1(?:0Over1|1Over1|2Over1|Over(?:2|3))|2Over1|3Over(?:1|2|4)|4Over1|5Over1|6Over1|7Over1|8Over1|9Over1|Linear))|i(?:neLevelControlClassID|stenbackControlClassID))|MuteControlClassID|O(?:bject(?:ClassID(?:Wildcard)?|Property(?:BaseClass|C(?:lass|ontrolList|reator)|Element(?:CategoryName|Master|N(?:ame|umberName)|Wildcard)|FirmwareVersion|Identify|Listener(?:Added|Removed)|M(?:anufacturer|odelName)|Name|Owne(?:dObjects|r)|S(?:cope(?:Global|Input|Output|PlayThrough|Wildcard)|e(?:lectorWildcard|rialNumber)))|SystemObject|Unknown)|fflineUnit(?:Property_(?:InputSize|OutputSize)|RenderAction_(?:Complete|Preflight|Render))|utputUnit(?:Property_(?:C(?:hannelMap|urrentDevice)|EnableIO|HasIO|IsRunning|S(?:etInputCallback|tartTime(?:stampsAtZero)?))|Range|St(?:artSelect|opSelect)))|P(?:ha(?:ntomPowerControlClassID|seInvertControlClassID)|lugIn(?:C(?:lassID|reateAggregateDevice)|DestroyAggregateDevice|Property(?:B(?:oxList|undleID)|ClockDeviceList|DeviceList|TranslateUIDTo(?:Box|ClockDevice|Device))|sFolderType)|r(?:esetsFolderType|opertyWildcard(?:Channel|PropertyID|Section)))|Queue(?:DeviceProperty_(?:NumberChannels|SampleRate)|Err_(?:Buffer(?:E(?:mpty|nqueuedTwice)|InQueue)|C(?:annotStart(?:Yet)?|odecNotFound)|DisposalPending|EnqueueDuringReset|Invalid(?:Buffer|CodecAccess|Device|OfflineMode|P(?:arameter|roperty(?:Size|Value)?)|QueueType|RunState|Tap(?:Context|Type))|P(?:ermissions|rimeTimedOut)|QueueInvalidated|RecordUnderrun|TooManyTaps)|P(?:aram_(?:P(?:an|itch|layRate)|Volume(?:RampTime)?)|ro(?:cessingTap_(?:EndOfStream|P(?:ostEffects|reEffects)|S(?:iphon|tartOfStream))|perty_(?:C(?:hannelLayout|onverterError|urrent(?:Device|LevelMeter(?:DB)?))|DecodeBufferSizeFrames|Enable(?:LevelMetering|TimePitch)|IsRunning|Ma(?:gicCookie|ximumOutputPacketSize)|StreamDescription|TimePitch(?:Algorithm|Bypass))))|TimePitchAlgorithm_(?:Spectral|TimeDomain|Varispeed))|S(?:e(?:lectorControl(?:ClassID|ItemKindSpacer|Property(?:AvailableItems|CurrentItem|Item(?:Kind|Name)))|rvices(?:Bad(?:PropertySizeError|SpecifierSizeError)|NoError|Property(?:CompletePlaybackIfAppDies|IsUISound)|SystemSound(?:ClientTimedOutError|ExceededMaximumDurationError|UnspecifiedError)|UnsupportedPropertyError)|ttingsFlags_(?:ExpertParameter|InvisibleParameter|MetaParameter|UserInterfaceParameter))|liderControl(?:ClassID|Property(?:Range|Value))|o(?:loControlClassID|und(?:BanksFolderType|sFolderType))|t(?:ereoPanControl(?:ClassID|Property(?:PanningChannels|Value))|ream(?:ClassID|Property(?:Available(?:PhysicalFormats|VirtualFormats)|Direction|IsActive|Latency|OwningDevice|PhysicalFormat(?:Match|Supported|s)?|StartingChannel|TerminalType|VirtualFormat)|TerminalType(?:Di(?:gitalAudioInterface|splayPort)|H(?:DMI|ead(?:phones|setMicrophone))|L(?:FESpeaker|ine)|Microphone|Receiver(?:Microphone|Speaker)|Speaker|TTY|Unknown)|Unknown))|u(?:bDevice(?:ClassID|DriftCompensation(?:HighQuality|LowQuality|M(?:axQuality|ediumQuality|inQuality))|Property(?:DriftCompensation(?:Quality)?|ExtraLatency))|pportFolderType)|ystemObjectClassID)|T(?:alkbackControlClassID|imeStamp(?:HostTimeValid|NothingValid|RateScalarValid|S(?:MPTETimeValid|ample(?:HostTimeValid|TimeValid))|WordClockTimeValid)|oolboxErr(?:_(?:CannotDoInCurrentContext|EndOfTrack|I(?:llegalTrackDestination|nvalid(?:EventType|PlayerState|SequenceType))|NoSequence|StartOfTrack|Track(?:IndexError|NotFound))|or_NoTrackDestination)|ransportManager(?:C(?:lassID|reateEndPointDevice)|DestroyEndPointDevice|Property(?:EndPointList|Trans(?:lateUIDToEndPoint|portType))))|Unit(?:Add(?:PropertyListenerSelect|RenderNotifySelect)|C(?:lumpID_System|omplexRenderSelect)|E(?:rr_(?:CannotDoInCurrentContext|ExtensionNotFound|F(?:ailedInitialization|ileNotSpecified|ormatNotSupported)|I(?:llegalInstrument|n(?:itialized|strumentTypeNotFound|valid(?:Element|File(?:Path)?|OfflineRender|P(?:arameter(?:Value)?|roperty(?:Value)?)|Scope)))|M(?:IDIOutputBufferFull|issingKey)|NoConnection|PropertyNot(?:InUse|Writable)|RenderTimeout|TooManyFramesToProcess|Un(?:authorized|initialized|knownFileType))|vent_(?:BeginParameterChangeGesture|EndParameterChangeGesture|P(?:arameterValueChange|ropertyChange)))|GetP(?:arameterSelect|roperty(?:InfoSelect|Select))|InitializeSelect|M(?:anufacturer_Apple|igrateProperty_(?:FromPlugin|OldAutomation))|OfflineProperty_(?:InputSize|OutputSize|Preflight(?:Name|Requirements)|StartOffset)|P(?:arameter(?:Flag_(?:C(?:FNameRelease|anRamp)|Display(?:Cube(?:Root|d)|Exponential|Logarithmic|Mask|Square(?:Root|d))|ExpertMode|G(?:lobal|roup)|Has(?:C(?:FNameString|lump)|Name)|I(?:nput|s(?:ElementMeta|GlobalMeta|HighResolution|Readable|Writable))|MeterReadOnly|NonRealTime|O(?:mitFromPresets|utput)|PlotHistory|ValuesHaveStrings)|Name_Full|Unit_(?:AbsoluteCents|B(?:PM|eats|oolean)|C(?:ents|ustomUnit)|De(?:cibels|grees)|EqualPowerCrossfade|Generic|Hertz|Indexed|LinearGain|M(?:IDI(?:Controller|NoteNumber)|eters|i(?:lliseconds|xerFaderCurve1))|Octaves|P(?:an|ercent|hase)|R(?:at(?:e|io)|elativeSemiTones)|S(?:ampleFrames|econds)))|ro(?:cess(?:MultipleSelect|Select)|perty_(?:A(?:UHostIdentifier|ddParameterMIDIMapping|llParameterMIDIMappings|udioChannelLayout)|B(?:usCount|ypassEffect)|C(?:PULoad|lassInfo(?:FromDocument)?|o(?:coaUI|ntextName)|urrentP(?:layTime|reset))|De(?:ferredRenderer(?:ExtraLatency|PullSize|WaitFrames)|pendentParameters)|Element(?:Count|Name)|F(?:a(?:ctoryPresets|stDispatch)|requencyResponse)|GetUIComponentList|Ho(?:stCallbacks|tMapParameterMIDIMapping)|I(?:conLocation|n(?:PlaceProcessing|put(?:AnchorTimeStamp|SamplesInOutput)))|La(?:stRenderError|tency)|M(?:IDI(?:ControlMapping|OutputCallback(?:Info)?)|a(?:keConnection|trix(?:Dimensions|Levels)|ximumFramesPerSlice)|eter(?:Clipping|ingMode))|NickName|OfflineRender|P(?:a(?:nnerMode|rameter(?:ClumpName|HistoryInfo|I(?:DName|nfo)|List|StringFromValue|Value(?:FromString|Name|Strings)|sForOverview))|resent(?:Preset|ationLatency))|Re(?:moveParameterMIDIMapping|nderQuality|questViewController|verbRoomType)|S(?:RCAlgorithm|ampleRate(?:ConverterComplexity)?|chedule(?:AudioSlice|StartTimeStamp|dFile(?:BufferSizeFrames|IDs|NumberBuffers|Prime|Region))|et(?:ExternalBuffer|RenderCallback)|houldAllocateBuffer|p(?:atial(?:Mixer(?:AttenuationCurve|DistanceParams|RenderingFlags)|izationAlgorithm)|e(?:akerConfiguration|echChannel))|treamFormat|upport(?:ed(?:ChannelLayoutTags|NumChannels)|sMPE))|TailTime|UsesInternalReverb|Voice)))|R(?:ange|e(?:move(?:PropertyListener(?:Select|WithUserDataSelect)|RenderNotifySelect)|nder(?:Action_(?:DoNotCheckRenderArgs|OutputIsSilence|P(?:ostRender(?:Error)?|reRender))|Select)|setSelect))|S(?:RCAlgorithm_(?:MediumQuality|Polyphase)|ampleRateConverterComplexity_(?:Linear|Mastering|Normal)|c(?:heduleParametersSelect|ope_(?:G(?:lobal|roup)|Input|Layer(?:Item)?|Note|Output|Part))|etP(?:arameterSelect|ropertySelect)|ubType_(?:A(?:U(?:Converter|Filter|iPodTimeOther)|udioFilePlayer)|BandPassFilter|D(?:LSSynth|e(?:f(?:aultOutput|erredRenderer)|lay)|istortion|ynamicsProcessor)|G(?:enericOutput|raphicEQ)|H(?:ALOutput|RTFPanner|igh(?:PassFilter|ShelfFilter))|Low(?:PassFilter|ShelfFilter)|M(?:IDISynth|atrix(?:Mixer|Reverb)|erger|ulti(?:BandCompressor|ChannelMixer|Splitter))|N(?:BandEQ|e(?:t(?:Receive|Send)|wTimePitch))|P(?:arametricEQ|eakLimiter|itch)|R(?:everb2|o(?:gerBeep|undTripAAC))|S(?:ample(?:Delay|r)|cheduledSoundPlayer|oundFieldPanner|p(?:atialMixer|eechSynthesis|hericalHeadPanner|litter)|tereoMixer|ystemOutput)|TimePitch|V(?:arispeed|ectorPanner|oiceProcessingIO)))|Type_(?:Effect|FormatConverter|Generator|M(?:IDIProcessor|ixer|usic(?:Device|Effect))|O(?:fflineEffect|utput)|Panner)|UninitializeSelect|yCodecComponentType)|V(?:STFolderType|olumeControlClassID)|_(?:BadFilePathError|File(?:NotFoundError|PermissionError)|MemFullError|ParamError|TooManyFilesOpenError|UnimplementedError))|t(?:h(?:TypeKCItemAttr|orizationFlag(?:CanNotPreAuthorize|De(?:faults|stroyRights)|ExtendRights|InteractionAllowed|NoData|P(?:artialRights|reAuthorize)))|o(?:GenerateReturnID|matorWorkflowsFolderType|saveInformationFolderType)))|vailBoundsChangedFor(?:D(?:isplay|ock)|MenuBar))|B(?:LibTag2|SLN(?:C(?:ontrolPointFormat(?:NoMap|WithMap)|urrentVersion)|DistanceFormat(?:NoMap|WithMap)|HangingBaseline|Ideographic(?:CenterBaseline|HighBaseline|LowBaseline)|LastBaseline|MathBaseline|N(?:oBaseline(?:Override)?|umBaselineClasses)|RomanBaseline|Tag)|T(?:B(?:adCloseMask|igKeysMask)|HeaderNode|IndexNode|LeafNode|MapNode|VariableIndexKeysMask)|a(?:ck(?:spaceCharCode|wardArrowIcon)|d(?:A(?:dapterErr|rg(?:LengthErr|sErr)|ttributeErr)|BaseErr|C(?:ISErr|ustomIFIDErr)|DeviceErr|EDCErr|HandleErr|IRQErr|LinkErr|OffsetErr|PageErr|S(?:izeErr|ocketErr|peedErr)|T(?:upleDataErr|ypeErr)|V(?:ccErr|ppErr)|WindowErr)|ndpassParam_(?:Bandwidth|CenterFrequency))|ellCharCode|ig5_(?:BasicVariant|DOSVariant|ETenVariant|StandardVariant)|lessed(?:BusErrorBait|Folder)|o(?:otTimeStartupItemsFolderType|xAnnotationSelector)|ridgeSoftwareRunningCantSleep|u(?:llet(?:CharCode|Unicode)|rningIcon|syErr|ttonDialogItem)|y(?:CommentView|DateView|IconView|KindView|LabelView|NameView|S(?:izeView|mallIcon)|VersionView|tePacketTranslationFlag_IsEstimate))|C(?:A(?:Clock(?:Message_(?:Armed|Disarmed|PropertyChanged|St(?:art(?:TimeSet|ed)|opped)|WrongSMPTEFormat)|Property_(?:InternalTimebase|M(?:IDIClockDestinations|TC(?:Destinations|FreewheelTime)|eterTrack)|Name|S(?:MPTE(?:Format|Offset)|endMIDISPP|ync(?:Mode|Source))|T(?:empoMap|imebaseSource))|SyncMode_(?:Internal|M(?:IDIClockTransport|TCTransport))|Time(?:Format_(?:AbsoluteSeconds|Beats|HostTime|S(?:MPTE(?:Seconds|Time)|amples|econds))|base_(?:Audio(?:Device|OutputUnit)|HostTime))|_(?:CannotSetTimeError|Invalid(?:P(?:layRateError|ropertySizeError)|S(?:MPTE(?:FormatError|OffsetError)|ync(?:ModeError|SourceError))|Time(?:FormatError|base(?:Error|SourceError))|UnitError)|UnknownPropertyError))|F(?:LinearPCMFormatFlagIs(?:Float|LittleEndian)|MarkerType_(?:Edit(?:Destination(?:Begin|End)|Source(?:Begin|End))|Generic|Index|KeySignature|Program(?:End|Start)|Re(?:gion(?:End|S(?:tart|yncPoint))|leaseLoop(?:End|Start))|S(?:avedPlayPosition|election(?:End|Start)|ustainLoop(?:End|Start))|T(?:empo|imeSignature|rack(?:End|Start)))|RegionFlag_(?:LoopEnable|Play(?:Backward|Forward))|_(?:AudioDataChunkID|ChannelLayoutChunkID|EditCommentsChunkID|F(?:il(?:e(?:Type|Version_Initial)|lerChunkID)|ormatListID)|In(?:foStringsChunkID|strumentChunkID)|M(?:IDIChunkID|a(?:gicCookieID|rkerChunkID))|OverviewChunkID|P(?:acketTableChunkID|eakChunkID)|RegionChunkID|S(?:MPTE_TimeType(?:2(?:398|4|5|997(?:Drop)?)|30(?:Drop)?|5(?:0|994(?:Drop)?)|60(?:Drop)?|None)|tr(?:eamDescriptionChunkID|ingsChunkID))|U(?:MIDChunkID|UIDChunkID)|iXMLChunkID)))|CRegister(?:CBit|NBit|VBit|XBit|ZBit)|F(?:Error(?:HTTP(?:AuthenticationTypeUnsupported|Bad(?:Credentials|ProxyCredentials|URL)|ConnectionLost|P(?:arseFailure|roxyConnectionFailure)|RedirectionLoopDetected|SProxyConnectionFailure)|PACFile(?:Auth|Error))|FTPErrorUnexpectedStatusCode|H(?:TTPCookieCannotParseCookieFile|ost(?:Addresses|Error(?:HostNotFound|Unknown)|Names|Reachability))|M68kRTA|NetService(?:Error(?:BadArgument|C(?:ancel|ollision)|DNSServiceFailure|In(?:Progress|valid)|NotFound|Timeout|Unknown)|Flag(?:IsD(?:efault|omain)|MoreComing|NoAutoRename|Remove)|MonitorTXT|sError(?:BadArgument|C(?:ancel|ollision)|In(?:Progress|valid)|NotFound|Timeout|Unknown))|S(?:OCKS(?:4Error(?:Id(?:Conflict|entdFailed)|RequestFailed|UnknownStatusCode)|5Error(?:Bad(?:Credentials|ResponseAddr|State)|NoAcceptableMethod|UnsupportedNegotiationMethod)|ErrorUn(?:knownClientVersion|supportedServerVersion))|treamError(?:HTTP(?:Authentication(?:Bad(?:Password|UserName)|TypeUnsupported)|BadURL|ParseFailure|RedirectionLoop|SProxyFailureUnexpectedResponseToCONNECTMethod)|SOCKS(?:4(?:Id(?:Conflict|entdFailed)|RequestFailed|SubDomainResponse)|5(?:Bad(?:ResponseAddr|State)|SubDomain(?:Method|Response|UserPass))|SubDomain(?:None|VersionCode)|UnknownClientVersion)))|URLError(?:AppTransportSecurityRequiresSecureConnection|Ba(?:ckgroundSession(?:InUseByAnotherProcess|WasDisconnected)|d(?:ServerResponse|URL))|C(?:a(?:llIsActive|n(?:celled|not(?:C(?:loseFile|onnectToHost|reateFile)|Decode(?:ContentData|RawData)|FindHost|LoadFromNetwork|MoveFile|OpenFile|ParseResponse|RemoveFile|WriteToFile)))|lientCertificateRe(?:jected|quired))|D(?:NSLookupFailed|ata(?:LengthExceedsMaximum|NotAllowed)|ownloadDecodingFailed(?:MidStream|ToComplete))|File(?:DoesNotExist|IsDirectory|OutsideSafeArea)|HTTPTooManyRedirects|InternationalRoamingOff|N(?:etworkConnectionLost|o(?:PermissionsToReadFile|tConnectedToInternet))|Re(?:directToNonExistentLocation|questBodyStreamExhausted|sourceUnavailable)|Se(?:cureConnectionFailed|rverCertificate(?:Has(?:BadDate|UnknownRoot)|NotYetValid|Untrusted))|TimedOut|U(?:n(?:known|supportedURL)|ser(?:AuthenticationRequired|CancelledAuthentication))|ZeroByteResource))|G(?:Image(?:AnimationStatus_(?:AllocationFailure|CorruptInputImage|IncompleteInputImage|ParameterError|UnsupportedFormat)|Metadata(?:Error(?:BadArgument|ConflictingArguments|PrefixConflict|Un(?:known|supportedFormat))|Type(?:A(?:lternate(?:Array|Text)|rray(?:Ordered|Unordered))|Default|Invalid|Str(?:ing|ucture)))|PropertyOrientation(?:Down(?:Mirrored)?|Left(?:Mirrored)?|Right(?:Mirrored)?|Up(?:Mirrored)?)|Status(?:Complete|In(?:complete|validData)|ReadingHeader|Un(?:expectedEOF|knownType)))|L(?:Bad(?:A(?:ddress|lloc|ttribute)|Co(?:deModule|n(?:nection|text))|D(?:isplay|rawable)|Enumeration|FullScreen|Match|OffScreen|P(?:ixelFormat|roperty)|RendererInfo|State|Value|Window)|C(?:E(?:CrashOnRemovedFunctions|DisplayListOptimization|MPEngine|Rasterization|S(?:tateValidation|urfaceBackingSize|wap(?:Limit|Rectangle)))|P(?:C(?:lientStorage|ontextPriorityRequest(?:High|Low|Normal)|urrentRendererID)|DispatchTableSize|GPU(?:FragmentProcessing|RestartStatus(?:Blacklisted|Caused|None)|VertexProcessing)|HasDrawable|MPSwapsInFlight|ReclaimResources|S(?:urface(?:BackingSize|O(?:pacity|rder)|SurfaceVolatile|Texture)|wap(?:Interval|Rectangle))))|GO(?:ClearFormatCache|FormatCacheSize|Re(?:setLibrary|tainRenderers)|Use(?:BuildCache|ErrorHandler))|NoError|OGLPVersion_(?:3_2_Core|GL3_Core|Legacy)|PFA(?:A(?:cc(?:elerated(?:Compute)?|umSize)|l(?:l(?:Renderers|owOfflineRenderers)|phaSize)|ux(?:Buffers|DepthStencil))|Backing(?:Store|Volatile)|C(?:losestPolicy|o(?:lor(?:Float|Size)|mpliant))|D(?:epthSize|isplayMask|oubleBuffer)|FullScreen|M(?:PSafe|aximumPolicy|inimumPolicy|ulti(?:Screen|sample))|NoRecovery|O(?:ffScreen|penGLProfile)|PBuffer|R(?:e(?:motePBuffer|ndererID)|obust)|S(?:ample(?:Alpha|Buffers|s)|ingleRenderer|te(?:ncilSize|reo)|upersample)|TripleBuffer|VirtualScreenCount|Window)|R(?:P(?:Acc(?:elerated(?:Compute)?|umModes)|B(?:ackingStore|ufferModes)|Co(?:lorModes|mpliant)|D(?:epthModes|isplayMask)|FullScreen|GPU(?:FragProcCapable|VertProcCapable)|M(?:PSafe|ax(?:AuxBuffers|Sample(?:Buffers|s))|ultiScreen)|O(?:ffScreen|nline)|R(?:enderer(?:Count|ID)|obust)|S(?:ample(?:Alpha|Modes)|tencilModes)|TextureMemory(?:Megabytes)?|VideoMemory(?:Megabytes)?|Window)|enderer(?:A(?:TIRa(?:deon(?:8500ID|9700ID|ID|X(?:1000ID|2000ID|3000ID))|ge(?:128ID|ProID))|ppleSWID)|Ge(?:Force(?:2MXID|3ID|8xxxID|FXID)|neric(?:FloatID|ID))|Intel(?:900ID|HD(?:4000ID|ID)|X3100ID)|Mesa3DFXID|VTBladeXP2ID))))|JK(?:ItalicRoman(?:O(?:ffSelector|nSelector)|Selector)|RomanSpacingType|SymbolAlt(?:F(?:iveSelector|ourSelector)|OneSelector|T(?:hreeSelector|woSelector)|ernativesType)|VerticalRoman(?:CenteredSelector|HBaselineSelector|PlacementType))|M(?:ActivateTextService|CopyTextServiceInputModeList|DeactivateTextService|F(?:ixTextService|loatBitmapFlags(?:Alpha(?:Premul)?|None|RangeClipped))|Get(?:InputModePaletteMenu|ScriptLangSupport|TextService(?:Menu|Property))|H(?:elpItem(?:AppleGuide|NoHelp|OtherHelp|RemoveHelp)|idePaletteWindows)|In(?:itiateTextService|putModePaletteItemHit)|MenuItemSelected|NothingSelected|S(?:Attr(?:Apple(?:CodesigningHashAgility(?:V2)?|ExpirationTime)|None|S(?:igningTime|mime(?:Capabilities|EncryptionKeyPrefs|MSEncryptionKeyPrefs)))|Certificate(?:Chain(?:WithRoot(?:OrFail)?)?|None|SignerOnly)|Signer(?:Invalid(?:Cert|Index|Signature)|NeedsDetachedContent|Unsigned|Valid)|etTextService(?:Cursor|Property)|howHelpSelected)|Te(?:rminateTextService|xtService(?:Event(?:Ref)?|MenuSelect))|UCTextServiceEvent)|S(?:Accept(?:AllComponentsMode|ThreadSafeComponentsOnlyMode)|DiskSpaceRecoveryOptionNoUI|Identity(?:AuthorityNotAccessibleErr|C(?:lass(?:Group|User)|ommitCompleted)|D(?:eletedErr|uplicate(?:FullNameErr|PosixNameErr))|Flag(?:Hidden|None)|Invalid(?:FullNameErr|PosixNameErr)|PermissionErr|Query(?:Event(?:ErrorOccurred|Results(?:Added|Changed|Removed)|SearchPhaseFinished)|GenerateUpdateEvents|IncludeHiddenIdentities|String(?:BeginsWith|Equals))|UnknownAuthorityErr)|SM_APPLEDL_MASK_MODE|tackBased)|T(?:CharacterCollection(?:Adobe(?:CNS1|GB1|Japan(?:1|2)|Korea1)|IdentityMapping)|F(?:ont(?:BoldTrait|C(?:la(?:rendonSerifsClass|ss(?:ClarendonSerifs|FreeformSerifs|M(?:ask(?:Shift|Trait)|odernSerifs)|O(?:ldStyleSerifs|rnamentals)|S(?:ansSerif|cripts|labSerifs|ymbolic)|TransitionalSerifs|Unknown))|o(?:l(?:lectionCopy(?:DefaultOptions|StandardSort|Unique)|orGlyphsTrait)|mpositeTrait|ndensedTrait))|DescriptorMatching(?:D(?:id(?:Begin|F(?:ailWithError|inish(?:Downloading)?)|Match)|ownloading)|Stalled|WillBegin(?:Downloading|Querying))|ExpandedTrait|F(?:ormat(?:Bitmap|OpenType(?:PostScript|TrueType)|PostScript|TrueType|Unrecognized)|reeformSerifsClass)|ItalicTrait|M(?:anager(?:AutoActivation(?:D(?:efault|isabled)|Enabled)|Error(?:A(?:lreadyRegistered|ssetNotFound)|CancelledByUser|DuplicatedName|ExceededResourceLimit|FileNotFound|In(?:Use|sufficient(?:Info|Permissions)|validF(?:ilePath|ontData))|MissingEntitlement|NotRegistered|RegistrationFailed|SystemRequired|UnrecognizedFormat)|Scope(?:None|P(?:ersistent|rocess)|Session|User))|o(?:dernSerifsClass|noSpaceTrait))|O(?:ldStyleSerifsClass|ptions(?:Default|Pre(?:ferSystemFont|ventAutoActivation))|r(?:ientation(?:Default|Horizontal|Vertical)|namentalsClass))|Priority(?:Computer|Dynamic|Network|Process|System|User)|S(?:ansSerifClass|criptsClass|labSerifsClass|ymbolicClass)|T(?:able(?:A(?:cnt|nkr|var)|B(?:ASE|dat|hed|loc|sln)|C(?:B(?:DT|LC)|FF(?:2)?|OLR|PAL|idg|map|v(?:ar|t))|DSIG|EB(?:DT|LC|SC)|F(?:dsc|eat|mtx|ond|pgm|var)|G(?:DEF|POS|SUB|asp|lyf|var)|H(?:VAR|dmx|ead|hea|mtx|sty)|J(?:STF|ust)|Ker(?:n|x)|L(?:TSH|car|oca|tag)|M(?:ATH|ERG|VAR|axp|eta|or(?:t|x))|Name|O(?:S2|p(?:bd|tionNoOptions))|P(?:CLT|ost|r(?:ep|op))|S(?:TAT|VG|bi(?:t|x))|Trak|V(?:DMX|ORG|VAR|hea|mtx)|Xref|Zapf)|ra(?:it(?:Bold|C(?:lassMask|o(?:lorGlyphs|mposite|ndensed))|Expanded|Italic|MonoSpace|UIOptimized|Vertical)|nsitionalSerifsClass))|U(?:I(?:Font(?:A(?:lertHeader|pplication)|ControlContent|EmphasizedSystem(?:Detail)?|Label|M(?:e(?:nu(?:Item(?:CmdKey|Mark)?|Title)|ssage)|ini(?:EmphasizedSystem|System))|None|P(?:alette|ushButton)|S(?:mall(?:EmphasizedSystem|System|Toolbar)|ystem(?:Detail)?)|Tool(?:Tip|bar)|U(?:ser(?:FixedPitch)?|tilityWindowTitle)|Views|WindowTitle)|OptimizedTrait)|nknownClass)|VerticalTrait)|rameP(?:athFill(?:EvenOdd|WindingNumber)|rogression(?:LeftToRight|RightToLeft|TopToBottom)))|Line(?:B(?:ounds(?:ExcludeTypographic(?:Leading|Shifts)|IncludeLanguageExtents|Use(?:GlyphPathBounds|HangingPunctuation|OpticalBounds))|reakBy(?:C(?:harWrapping|lipping)|Truncating(?:Head|Middle|Tail)|WordWrapping))|Truncation(?:End|Middle|Start))|ParagraphStyleSpecifier(?:Alignment|BaseWritingDirection|Count|DefaultTabInterval|FirstLineHeadIndent|HeadIndent|Line(?:B(?:oundsOptions|reakMode)|HeightMultiple|SpacingAdjustment)|M(?:aximumLine(?:Height|Spacing)|inimumLine(?:Height|Spacing))|ParagraphSpacing(?:Before)?|Ta(?:bStops|ilIndent))|Run(?:Delegate(?:CurrentVersion|Version1)|Status(?:HasNonIdentityMatrix|No(?:Status|nMonotonic)|RightToLeft))|TextAlignment(?:Center|Justified|Left|Natural|Right)|Underline(?:Pattern(?:D(?:ash(?:Dot(?:Dot)?)?|ot)|Solid)|Style(?:Double|None|Single|Thick))|WritingDirection(?:Embedding|LeftToRight|Natural|Override|RightToLeft))|UPSPPDDomain|V(?:AttachmentMode_Should(?:NotPropagate|Propagate)|Pixel(?:Buffer(?:Lock_ReadOnly|PoolFlushExcessBuffers)|FormatType_(?:1(?:28RGBAFloat|4Bayer_(?:BGGR|G(?:BRG|RBG)|RGGB)|6(?:BE5(?:55|65)|Gray|LE5(?:55(?:1)?|65))|IndexedGray_WhiteIsZero|Monochrome)|2(?:4(?:BGR|RGB)|Indexed(?:Gray_WhiteIsZero)?)|3(?:0RGB(?:LEPackedWideGamut)?|2(?:A(?:BGR|RGB|lphaGray)|BGRA|RGBA))|4(?:2(?:0YpCbCr(?:10BiPlanar(?:FullRange|VideoRange)|8(?:BiPlanar(?:FullRange|VideoRange)|Planar(?:FullRange)?|VideoRange_8A_TriPlanar))|2YpCbCr(?:1(?:0(?:BiPlanar(?:FullRange|VideoRange))?|6)|8(?:FullRange|_yuvs)?|_4A_8BiPlanar))|44(?:4(?:AYpCbCr(?:16|8)|YpCbCrA8(?:R)?)|YpCbCr(?:10(?:BiPlanar(?:FullRange|VideoRange))?|8))|8RGB|Indexed(?:Gray_WhiteIsZero)?)|64(?:ARGB|RGBAHalf)|8Indexed(?:Gray_WhiteIsZero)?|ARGB2101010LEPacked|D(?:epthFloat(?:16|32)|isparityFloat(?:16|32))|OneComponent(?:16Half|32Float|8)|TwoComponent(?:16Half|32Float|8)))|Return(?:AllocationFailed|DisplayLink(?:AlreadyRunning|CallbacksNotSet|NotRunning)|Error|First|Invalid(?:Argument|Display|P(?:ixel(?:BufferAttributes|Format)|oolAttributes)|Size)|Last|P(?:ixelBufferNot(?:MetalCompatible|OpenGLCompatible)|oolAllocationFailed)|Retry|Success|Unsupported|WouldExceedAllocationThreshold)|SMPTETime(?:Running|Type(?:2(?:4|5|997(?:Drop)?)|30(?:Drop)?|5994|60)|Valid)|Time(?:IsIndefinite|Stamp(?:BottomField|HostTimeValid|IsInterlaced|RateScalarValid|SMPTETimeValid|TopField|Video(?:HostTimeValid|RefreshPeriodValid|TimeValid))))|a(?:chedDataFolderType|l(?:ibratorNamePrefix|lingConvention(?:Mask|Phase|Width))|n(?:onicalCompositionO(?:ffSelector|nSelector)|t(?:ConfigureCardErr|ReportProcessorTemperatureErr))|r(?:bonLibraryFolderType|d(?:BusCardErr|PowerOffErr))|seSensitive(?:Layout(?:O(?:ffSelector|nSelector)|Type)|SpacingO(?:ffSelector|nSelector))|utionIcon)|e(?:nterOn(?:MainScreen|Screen)|rt(?:Search(?:Any|Decrypt(?:Allowed|Disallowed|Ignored|Mask)|Encrypt(?:Allowed|Disallowed|Ignored|Mask)|PrivKeyRequired|S(?:hift|igning(?:Allowed|Disallowed|Ignored|Mask))|Unwrap(?:Allowed|Disallowed|Ignored|Mask)|Verify(?:Allowed|Disallowed|Ignored|Mask)|Wrap(?:Allowed|Disallowed|Ignored|Mask))|Usage(?:AllAdd|DecryptA(?:dd|skAndAdd)|EncryptA(?:dd|skAndAdd)|KeyExchA(?:dd|skAndAdd)|RootA(?:dd|skAndAdd)|S(?:SLA(?:dd|skAndAdd)|hift|igningA(?:dd|skAndAdd))|VerifyA(?:dd|skAndAdd))|ificateKCItemClass))|h(?:aracter(?:AlternativesType|PaletteInputMethodClass|ShapeType)|e(?:ck(?:BoxDialogItem|CharCode|Unicode)|wableItemsFolderType))|ircleAnnotationSelector|l(?:ass(?:KCItemAttr|ic(?:D(?:esktopFolderType|omain)|PreferencesFolderType))|ea(?:nUpAEUT|rCharCode)|i(?:entRequestDenied|p(?:boardIcon|ping(?:Creator|PictureType(?:Icon)?|SoundType(?:Icon)?|TextType(?:Icon)?|UnknownType(?:Icon)?))))|o(?:l(?:l(?:ate(?:AttributesNotFoundErr|BufferTooSmall|Invalid(?:C(?:har|ollationRef)|Options)|MissingUnicodeTableErr|PatternNotFoundErr|UnicodeConvertFailedErr)|ection(?:AllAttributes|D(?:efaultAttributes|ontWant(?:Attributes|Data|I(?:d|ndex)|Size|Tag))|Lock(?:Bit|Mask)|NoAttributes|Persistence(?:Bit|Mask)|Reserved(?:0(?:Bit|Mask)|1(?:0(?:Bit|Mask)|1(?:Bit|Mask)|2(?:Bit|Mask)|3(?:Bit|Mask)|Bit|Mask)|2(?:Bit|Mask)|3(?:Bit|Mask)|4(?:Bit|Mask)|5(?:Bit|Mask)|6(?:Bit|Mask)|7(?:Bit|Mask)|8(?:Bit|Mask)|9(?:Bit|Mask))|User(?:0(?:Bit|Mask)|1(?:0(?:Bit|Mask)|1(?:Bit|Mask)|2(?:Bit|Mask)|3(?:Bit|Mask)|4(?:Bit|Mask)|5(?:Bit|Mask)|Bit|Mask)|2(?:Bit|Mask)|3(?:Bit|Mask)|4(?:Bit|Mask)|5(?:Bit|Mask)|6(?:Bit|Mask)|7(?:Bit|Mask)|8(?:Bit|Mask)|9(?:Bit|Mask)|Attributes)))|or(?:Picker(?:AppIsColorSyncAware|Ca(?:llColorProcLive|n(?:AnimatePalette|ModifyPalette))|D(?:etachedFromChoices|ialogIsMo(?:dal|veable))|In(?:ApplicationDialog|PickerDialog|SystemDialog)|sFolderType)|Sync(?:1(?:0BitInteger|6Bit(?:Float|Integer)|BitGamut)|32Bit(?:Float|Integer|NamedColorIndex)|8BitInteger|Alpha(?:First|InfoMask|Last|None(?:Skip(?:First|Last))?|Premultiplied(?:First|Last))|ByteOrder(?:16(?:Big|Little)|32(?:Big|Little)|Default|Mask)|CMMFolderType|Folder(?:Icon|Type)|ProfilesFolderType|ScriptingFolderType))?)|m(?:m(?:and(?:CharCode|Unicode)|entKCItemAttr|on(?:LigaturesO(?:ffSelector|nSelector)|NameKCItemAttr))|p(?:atibilityCompositionO(?:ffSelector|nSelector)|o(?:nent(?:AliasResourceType|C(?:anDoSelect|loseSelect)|DebugOption|ExecuteWiredActionSelect|Get(?:MPWorkFunctionSelect|PublicResourceSelect)|OpenSelect|Re(?:gisterSelect|sourceType)|TargetSelect|UnregisterSelect|VersionSelect|sFolderType)|sitionsFolderType)|uterIcon))|n(?:figurationLockedErr|n(?:Suite|ect(?:ToIcon|ion(?:AudioStreaming|BlueGammaScale|C(?:h(?:anged|eckEnable)|o(?:lor(?:DepthsSupported|Mode(?:sSupported)?)|ntroller(?:ColorDepth|D(?:epthsSupported|itherControl))))|Display(?:Flags|Parameter(?:Count|s))|Enable(?:Audio)?|Fl(?:ags|ushParameters)|G(?:ammaScale|reenGammaScale)|HandleDisplayPortEvent|Ignore|Overscan|P(?:anelTimingDisable|o(?:stWake|wer)|robe)|RedGammaScale|S(?:tartOfFrameTime|upports(?:AppleSense|HLDDCSense|LLDDCSense)|ync(?:Enable|Flags))|V(?:BLMultiplier|ideoBest))))|t(?:ainer(?:AliasType|CDROMAliasType|F(?:loppyAliasType|olderAliasType)|HardDiskAliasType|ServerAliasType|TrashAliasType)|extual(?:Alternates(?:O(?:ffSelector|nSelector)|Type)|LigaturesO(?:ffSelector|nSelector)|MenuItemsFolder(?:Icon|Type)|SwashAlternatesO(?:ffSelector|nSelector))|rol(?:A(?:dd(?:FontSizeMask|ToMetaFontMask)|utoToggles)|B(?:e(?:havior(?:CommandMenu|MultiValueMenu|OffsetContents|Pushbutton|S(?:ingleValueMenu|ticky)|Toggles)|velButton(?:Align(?:Bottom(?:Left|Right)?|Center|Left|Right|SysDirection|T(?:ext(?:Center|Flush(?:Left|Right)|SysDirection)|op(?:Left|Right)?))|C(?:enterPopupGlyphTag|ontentTag)|Graphic(?:AlignTag|OffsetTag)|IsMultiValueMenuTag|KindTag|La(?:rgeBevel(?:Proc|Variant)?|stMenuTag)|Menu(?:DelayTag|HandleTag|On(?:Bottom|Right(?:Variant)?)|RefTag|ValueTag)|NormalBevel(?:Proc|Variant)?|OwnedMenuRefTag|Place(?:AboveGraphic|BelowGraphic|Normally|SysDirection|To(?:LeftOfGraphic|RightOfGraphic))|S(?:caleIconTag|mallBevel(?:Proc|Variant)?)|T(?:ext(?:AlignTag|OffsetTag|PlaceTag)|ransformTag)))|oundsChange(?:PositionChanged|SizeChanged)|uttonPart)|C(?:h(?:asingArrows(?:AnimatingTag|Proc)|eckBox(?:AutoToggleProc|CheckedValue|MixedValue|P(?:art|roc)|UncheckedValue))|l(?:ickableMetaPart|ock(?:A(?:MPMPart|bsoluteTimeTag|nimatingTag)|DateProc|F(?:lag(?:DisplayOnly|Live|Standard)|ontStyleTag)|HourDayPart|Is(?:DisplayOnly|Live)|LongDateTag|M(?:inuteMonthPart|onthYearProc)|NoFlags|Part|SecondYearPart|T(?:ime(?:Proc|SecondsProc)|ype(?:HourMinute(?:Second)?|Month(?:DayYear|Year)))))|o(?:l(?:lectionTag(?:Bounds|Command|ID(?:ID|Signature)|M(?:aximum|inimum)|RefCon|Title|UnicodeTitle|V(?:a(?:lue|rCode)|i(?:ewSize|sibility)))|orTableResourceType)|ntent(?:AlertIconRes|C(?:GImageRef|Icon(?:Handle|Res))|I(?:CON(?:Res)?|con(?:Ref|Suite(?:Handle|Res)))|MetaPart|Pict(?:Handle|Res)|T(?:ag|extOnly))))|D(?:ataBrowser(?:DraggedPart|EditText(?:KeyFilterTag|ValidationProcTag)|IncludesFrameAndFocusTag|KeyFilterTag|Part)|efProc(?:ResourceType|Type)|i(?:alogItem|s(?:abledPart|closure(?:Button(?:Closed|Disclosed)|TrianglePoint(?:Default|Left|Right))))|ownButtonPart)|E(?:dit(?:Text(?:C(?:FStringTag|harCount)|FixedTextTag|In(?:line(?:InputProc|P(?:ostUpdateProcTag|reUpdateProcTag))|sert(?:CFStringRefTag|TextBufferTag))|Key(?:FilterTag|ScriptBehaviorTag)|LockedTag|P(?:a(?:rt|ssword(?:CFStringTag|Proc|Tag))|roc)|S(?:electionTag|ingleLineTag|pellCheck(?:AsYouTypeTag|ingTag)|tyleTag)|T(?:EHandleTag|extTag)|ValidationProcTag)|UnicodeTextP(?:asswordProc|ostUpdateProcTag|roc))|ntireControl)|Fo(?:cus(?:N(?:extPart|oPart)|PrevPart)|nt(?:BigSystemFont|MiniSystemFont|S(?:mall(?:BoldSystemFont|SystemFont)|tyleTag)|ViewSystemFont))|G(?:etsFocusOnClick|roupBox(?:CheckBoxProc|F(?:ontStyleTag|rameRectTag)|Menu(?:HandleTag|RefTag)|PopupButtonProc|Secondary(?:CheckBoxProc|PopupButtonProc|TextTitleProc)|T(?:extTitleProc|itleRectTag)))|Ha(?:ndlesTracking|s(?:RadioBehavior|SpecialBackground))|I(?:con(?:AlignmentTag|ContentTag|NoTrackProc|P(?:art|roc)|Re(?:f(?:NoTrackProc|Proc)|sourceIDTag)|Suite(?:NoTrackProc|Proc)|TransformTag)|dlesWithTimer|mageWell(?:ContentTag|IsDragDestinationTag|P(?:art|roc)|TransformTag)|n(?:activePart|dicatorPart|vertsUpDownValueMeaning))|K(?:ey(?:Filter(?:BlockKey|PassKey|Tag)|ScriptBehavior(?:AllowAnyScript|PrefersRoman|RequiresRoman))|ind(?:BevelButton|C(?:h(?:asingArrows|eck(?:Box|GroupBox))|lock)|D(?:ataBrowser|isclosure(?:Button|Triangle))|Edit(?:Text|UnicodeText)|GroupBox|HI(?:ComboBox|GrowBoxView|ImageView|MenuView|S(?:crollView|earchField|tandardMenuView)|TextView)|I(?:con|mageWell)|Li(?:stBox|ttleArrows)|P(?:icture|lacard|opup(?:Arrow|Button|GroupBox)|rogressBar|ush(?:Button|IconButton))|R(?:adio(?:Button|Group)|elevanceBar|oundButton)|S(?:croll(?:Bar|ingTextBox)|eparator|ignatureApple|lider|taticText)|Ta(?:bs|g)|UserPane|WindowHeader))|L(?:abelPart|i(?:st(?:Box(?:AutoSizeProc|DoubleClick(?:Part|Tag)|FontStyleTag|KeyFilterTag|L(?:DEFTag|istHandleTag)|P(?:art|roc))|DescResType)|ttleArrows(?:IncrementValueTag|Proc)))|MenuPart|No(?:Content|Part|Variant)|OpaqueMetaPart|P(?:a(?:ge(?:DownPart|UpPart)|nel(?:DisabledFolder(?:Icon|Type)|Folder(?:AliasType|Icon(?:Resource)?|Type)))|icture(?:HandleTag|NoTrackProc|P(?:art|roc))|lacardProc|opup(?:Arrow(?:EastProc|NorthProc|Orientation(?:East|North|South|West)|S(?:ize(?:Normal|Small)|mall(?:EastProc|NorthProc|SouthProc|WestProc)|outhProc)|WestProc)|Button(?:CheckCurrentTag|ExtraHeightTag|Menu(?:HandleTag|IDTag|RefTag)|OwnedMenuRefTag|Proc)|FixedWidthVariant|Use(?:AddResMenuVariant|WFontVariant)|VariableWidthVariant)|ro(?:gressBar(?:AnimatingTag|IndeterminateTag|Proc)|pertyPersistent)|ushBut(?:LeftIconProc|RightIconProc|ton(?:AnimatingTag|C(?:ancelTag|ontentTag)|DefaultTag|I(?:con(?:AlignmentTag|On(?:Left|Right))|sTexturedTag)|Proc)))|R(?:adio(?:Button(?:AutoToggleProc|CheckedValue|MixedValue|P(?:art|roc)|UncheckedValue)|GroupP(?:art|roc))|elevanceBarProc|oundButton(?:ContentTag|LargeSize|NormalSize|SizeTag))|S(?:croll(?:Bar(?:LiveProc|Proc|ShowsArrowsTag)|TextBox(?:A(?:nimatingTag|utoScroll(?:AmountTag|Proc))|ContentsTag|DelayBe(?:foreAutoScrollTag|tweenAutoScrollTag)|Proc))|e(?:archField(?:CancelPart|MenuPart)|paratorLineProc)|ize(?:Auto|Large|Mini|Normal|Small|Tag)|lider(?:DoesNotPoint|HasTickMarks|LiveFeedback|NonDirectional|P(?:oints(?:DownOrRight|UpOrLeft)|roc)|ReverseDirection)|t(?:aticText(?:CFStringTag|IsMultilineTag|Proc|StyleTag|T(?:ext(?:HeightTag|Tag)|runcTag))|r(?:ipModulesFolder(?:Icon|Type)|uctureMetaPart))|upports(?:C(?:alcBestRect|lickActivation|ontextualMenus)|D(?:ataAccess|ragAndDrop)|Embedding|F(?:lattening|ocus)|G(?:etRegion|hosting)|LiveFeedback|SetCursor))|T(?:ab(?:ContentRectTag|Direction(?:East|North|South|West)|EnabledFlagTag|FontStyleTag|I(?:mageContentTag|nfo(?:Tag|Version(?:One|Zero)))|L(?:arge(?:EastProc|NorthProc|Proc|SouthProc|WestProc)|istResType)|S(?:ize(?:Large|Mini|Small)|mall(?:EastProc|NorthProc|Proc|SouthProc|WestProc)))|emplateResourceType|hemeText(?:FontTag|HorizontalFlushTag|InfoTag|TruncationTag|VerticalFlushTag)|riangle(?:AutoToggleProc|L(?:astValueTag|eftFacing(?:AutoToggleProc|Proc))|P(?:art|roc)))|U(?:nicode|pButtonPart|se(?:AllMask|BackColorMask|F(?:aceMask|o(?:ntMask|reColorMask))|JustMask|ModeMask|SizeMask|ThemeFontIDMask|r(?:ItemDrawProcTag|Pane(?:ActivateProcTag|BackgroundProcTag|DrawProcTag|FocusProcTag|HitTestProcTag|IdleProcTag|KeyDownProcTag|Proc|TrackingProcTag))|sOwningWindowsFontVariant))|W(?:ants(?:Activate|Idle)|indow(?:Header(?:IsListHeaderTag|Proc)|ListViewHeaderProc))))|verterPrimeMethod_(?:No(?:ne|rmal)|Pre))|operativeThread|re(?:E(?:ndian(?:AppleEventManagerDomain|ResourceManagerDomain)|ventClass)|ServicesFolderType))|reat(?:e(?:Folder(?:AtBoot(?:Bit)?)?|IfNeeded)|ionDateKCItemAttr|orKCItemAttr)|u(?:r(?:rent(?:MixedModeStateRecord|Process|ThreadID|User(?:Folder(?:Location|Type)|RemoteFolder(?:Location|Type)))|sive(?:ConnectionType|Selector))|stom(?:BadgeResource(?:ID|Type|Version)|Icon(?:KCItemAttr|Resource))))|D(?:0Dispatched(?:CStackBased|PascalStackBased)|1DispatchedPascalStackBased|CM(?:A(?:llowListing|nyFieldT(?:ag|ype))|BasicDictionaryClass|Can(?:AddDictionaryFieldMask|CreateDictionaryMask|HaveMultipleIndexMask|ModifyDictionaryMask|StreamDictionaryMask|Use(?:FileDictionaryMask|MemoryDictionaryMask|TransactionMask))|DictionaryHeader(?:Signature|Version)|Fi(?:ndMethod(?:B(?:ackwardTrie|eginningMatch)|ContainsMatch|E(?:ndingMatch|xactMatch)|ForwardTrie)|xedSizeFieldMask)|HiddenFieldMask|I(?:dentifyFieldMask|ndexedFieldMask)|Japanese(?:AccentT(?:ag|ype)|FukugouInfoT(?:ag|ype)|H(?:inshiT(?:ag|ype)|yokiT(?:ag|ype))|OnKunReadingT(?:ag|ype)|PhoneticT(?:ag|ype)|WeightT(?:ag|ype)|YomiT(?:ag|ype))|ProhibitListing|Re(?:ad(?:OnlyDictionary|WriteDictionary)|quiredFieldMask)|SpecificDictionaryClass|UserDictionaryClass)|M(?:CantBlock|D(?:isplay(?:AlreadyInstalledErr|NotFoundErr)|riverNotDisplayMgrAwareErr)|FoundErr|GenErr|M(?:ainDisplayCannotMoveErr|irroring(?:Blocked|NotOn|OnAlready))|No(?:DeviceTableclothErr|tFoundErr)|SWNotInitializedErr|WrongNumberOfDisplays)|OSJapanese(?:PalmVariant|StandardVariant)|R(?:AudioFileNotSupportedErr|B(?:adLayoutErr|lock(?:Size(?:Audio|DVDData|Mode(?:1Data|2(?:Data|Form(?:1Data|2Data))))|Type(?:Audio|DVDData|Mode(?:1Data|2(?:Data|Form(?:1Data|2Data)))))|urn(?:MediaWriteFailureErr|NotAllowedErr|PowerCalibrationErr|UnderrunErr))|CDText(?:Encoding(?:ASCII|ISOLatin1Modified)|GenreCode(?:A(?:dultContemporary|lternativeRock)|C(?:hildrens|lassical|o(?:ntemporaryChristian|untry))|Dance|E(?:asyListening|rotic)|Folk|Gospel|HipHop|Jazz|Latin|Musical|NewAge|Oper(?:a|etta)|Pop|R(?:ap|eggae|hythmAndBlues|ock)|S(?:ound(?:Effects|track)|pokenWord)|Unknown|WorldMusic))|D(?:ata(?:Form(?:Audio|DVDData|Mode(?:1Data|2(?:Data|Form(?:1Data|2Data))))|ProductionErr)|evice(?:AccessErr|Bu(?:rnStrategyNotAvailableErr|syErr)|C(?:antWrite(?:CDTextErr|I(?:SRCErr|ndexPointsErr)|SCMSErr)|ommunicationErr)|InvalidErr|Not(?:ReadyErr|SupportedErr)|PreGapLengthNotValidErr)|oubleLayerL0(?:AlreadySpecifiedErr|DataZoneBlocksParamErr))|F(?:i(?:le(?:Fork(?:Data|Resource|Size(?:Actual|Estimate))|LocationConflictErr|M(?:essage(?:ForkSize|P(?:ostBurn|r(?:eBurn|oduceData))|Release|VerificationStarting)|odifiedDuringBurnErr)|system(?:Mask(?:Default|HFSPlus|ISO9660|Joliet|UDF)|sNotSupportedErr))|rstErr)|lag(?:NoMoreData|SubchannelDataRequested)|unctionNotSupportedErr)|In(?:ternalErr|validIndexPointsErr)|LinkType(?:FinderAlias|HardLink|SymbolicLink)|Media(?:BusyErr|InvalidErr|Not(?:BlankErr|ErasableErr|PresentErr|SupportedErr|WritableErr))|S(?:essionFormat(?:Audio|CD(?:I|XA)|DVDData|Mode1Data)|peedTestAlreadyRunningErr)|T(?:ooMany(?:NameConflictsErr|TracksForDVDErr)|rack(?:M(?:essage(?:EstimateLength|P(?:ostBurn|r(?:eBurn|oduce(?:Data|PreGap)))|Verif(?:ication(?:Done|Starting)|y(?:Data|PreGap)))|ode(?:1Data|2(?:Data|Form(?:1Data|2Data))|Audio|DVDData))|ReusedErr))|UserCanceledErr|VerificationFailedErr)|Sp(?:Con(?:firmSwitchWarning|text(?:AlreadyReservedErr|Not(?:FoundErr|ReservedErr)))|FrameRateNotReadyErr|In(?:ternalErr|valid(?:AttributesErr|ContextErr))|NotInitializedErr|S(?:tereoContextErr|ystemSWTooOldErr))|TP(?:AbortJobErr|HoldJobErr|StopQueueErr|T(?:hirdPartySupported|ryAgainErr))|ata(?:A(?:ccessKCEvent(?:Mask)?|lignmentException)|Br(?:eakpointException|owser(?:A(?:lwaysExtendSelection|ttribute(?:AutoHideScrollBars|ColumnViewResizeWindow|ListView(?:AlternatingRowColors|DrawColumnDividers)|None|ReserveGrowBoxSpace))|C(?:heckboxT(?:riState|ype)|lientPropertyFlags(?:Mask|Offset)|mdTogglesSelection|o(?:lumnView(?:PreviewProperty)?|nt(?:ainer(?:AliasIDProperty|Clos(?:ed|ing)|Is(?:ClosableProperty|Open(?:ableProperty)?|SortableProperty)|Opened|Sort(?:ed|ing))|entHit))|ustomType)|D(?:ateTime(?:DateOnly|Relative|SecondsToo|T(?:imeOnly|ype))|efaultPropertyFlags|oNotTruncateText|ragSelect)|Edit(?:Msg(?:C(?:lear|opy|ut)|Paste|Redo|SelectAll|Undo)|St(?:arted|opped))|I(?:con(?:AndTextType|Type)|tem(?:A(?:dded|nyState)|D(?:eselected|oubleClicked)|Is(?:ActiveProperty|ContainerProperty|DragTarget|EditableProperty|Select(?:ableProperty|ed))|No(?:Property|State)|ParentContainerProperty|Removed|Sel(?:ected|fIdentityProperty)|s(?:A(?:dd|ssign)|Remove|Toggle)))|L(?:atestC(?:allbacks|ustomCallbacks)|istView(?:AppendColumn|DefaultColumnFlags|LatestHeaderDesc|MovableColumn|NoGapForIconInHeaderButton|S(?:electionColumn|ortableColumn)|TypeSelectColumn)?)|Metric(?:CellContentInset|Disclosure(?:Column(?:EdgeInset|PerDepthGap)|TriangleAndContentGap)|IconAndTextGap|Last)|N(?:everEmptySelectionSet|o(?:DisjointSelection|Item|View|thingHit))|Order(?:Decreasing|Increasing|Undefined)|P(?:opupMenu(?:Buttonless|Type)|ro(?:gressBarType|perty(?:C(?:heckboxPart|ontentPart)|DisclosurePart|EnclosingPart|Flags(?:Mask|Offset)|I(?:conPart|s(?:Editable|Mutable))|ModificationFlags|ProgressBarPart|RelevanceRankPart|SliderPart|TextPart)))|Re(?:l(?:ativeDateTime|evanceRankType)|setSelection|veal(?:AndCenterInView|Only|WithoutSelecting))|S(?:elect(?:OnlyOne|ion(?:Anchor(?:Down|Left|Right|Up)|SetChanged))|lider(?:DownwardThumb|PlainThumb|Type|UpwardThumb)|topTracking)|T(?:a(?:bleView(?:FillHilite|LastColumn|MinimalHilite|SelectionColumn)|rgetChanged)|extType|runcateText(?:At(?:End|Start)|Middle))|U(?:niversalPropertyFlags(?:Mask)?|ser(?:StateChanged|ToggledContainer))|ViewSpecific(?:Flags(?:Mask|Offset)|PropertyFlags))))|e(?:c(?:o(?:mposeDiacriticsSelector|rativeBordersSelector)|ryptKCItemAttr)|epestColorScreen|fault(?:C(?:JKRomanSelector|MMSignature|hangedKCEvent(?:Mask)?|olorPicker(?:Height|Width))|LowerCaseSelector|UpperCaseSelector)|l(?:ayParam_(?:DelayTime|Feedback|LopassCutoff|WetDryMix)|ete(?:AliasIcon|CharCode|KCEvent(?:Mask)?))|s(?:criptionKCItemAttr|ign(?:ComplexityType|Level(?:1Selector|2Selector|3Selector|4Selector|5Selector))|ktop(?:FolderType|Icon(?:Resource)?|P(?:icturesFolderType|rinterAliasType)))|v(?:eloper(?:ApplicationsFolderType|DocsFolderType|FolderType|HelpFolderType)|ice(?:InitiatedWake|ToPCS)))|i(?:a(?:criticsType|gonalFractionsSelector|l(?:ectBundleResType|og(?:F(?:lags(?:HandleMovableModal|Use(?:Co(?:mpositing|ntrolHierarchy)|Theme(?:Background|Controls)))|ont(?:Add(?:FontSizeMask|ToMetaFontMask)|NoFontStyle|Use(?:AllMask|BackColorMask|F(?:aceMask|o(?:nt(?:Mask|NameMask)|reColorMask))|JustMask|ModeMask|SizeMask|ThemeFontIDMask)))|WindowKind))|mond(?:AnnotationSelector|CharCode|Unicode))|ctionar(?:iesFolderType|yFileType)|giHub(?:Blank(?:CD|DVD)|EventClass|MusicCD|PictureCD|VideoDVD)|ngbatsSelector|phthongLigaturesO(?:ffSelector|nSelector)|rectoryServices(?:FolderType|PlugInsFolderType)|s(?:p(?:atched(?:ParameterPhase|SelectorSize(?:Phase|Width))|lay(?:ExtensionsFolderType|Mode(?:A(?:cceleratorBackedFlag|lwaysShowFlag)|BuiltInFlag|DefaultFlag|InterlacedFlag|N(?:ativeFlag|everShowFlag|ot(?:GraphicsQualityFlag|PresetFlag|ResizeFlag))|RequiresPanFlag|S(?:afe(?:Flag|tyFlags)|imulscanFlag|tretchedFlag)|TelevisionFlag|Valid(?:F(?:lag|or(?:AirPlayFlag|HiResFlag|MirroringFlag))|ateAgainstDisplay))|ProductIDGeneric|SubPixel(?:Configuration(?:Delta|Quad|Stripe(?:Offset)?|Undefined)|Layout(?:BGR|QuadGB(?:L|R)|RGB|Undefined)|Shape(?:Elliptical|Oval|R(?:ectangular|ound)|Square|Undefined))|TextSelector|VendorIDUnknown))|tortionParam_(?:CubicTerm|De(?:c(?:ay|imation(?:Mix)?)|lay(?:Mix)?)|FinalMix|LinearTerm|PolynomialMix|R(?:ingMod(?:Balance|Freq(?:1|2)|Mix)|ounding)|S(?:oftClipGain|quaredTerm)))|therAlgorithm_(?:NoiseShaping|TPDF))|o(?:FolderActionEvent|NotActivateAnd(?:HandleClick|IgnoreClick)|cument(?:Window(?:Class|VariantCode)|ationFolderType|sFolder(?:Icon|Type))|main(?:LibraryFolderType|TopLevelFolderType)|nt(?:CreateFolder|FindAppBySignature|PassSelector)|wn(?:ArrowCharCode|loadsFolderType)|ze(?:Demand|Request|ToFullWakeUp|WakeUp))|r(?:a(?:g(?:Action(?:Al(?:ias|l)|Copy|Delete|Generic|Move|Nothing|Private)|Behavior(?:None|ZoomBackAnimation)|D(?:ark(?:Translucency|erTranslucency)|oNotScaleImage)|FlavorType(?:HFS|PromiseHFS)|HasLeftSenderWindow|InsideSender(?:Application|Window)|OpaqueTranslucency|P(?:romisedFlavor(?:FindFile)?|seudo(?:CreatorVolumeOrDirectory|FileType(?:Directory|Volume)))|Region(?:AndImage|Begin|Draw|End|Hide|Idle)|Standard(?:DropLocation(?:Trash|Unknown)|Translucency)|Tracking(?:Enter(?:Control|Handler|Window)|In(?:Control|Window)|Leave(?:Control|Handler|Window)))|w(?:Control(?:EntireControl|IndicatorOnly)|erWindowClass))|op(?:BoxFolderType|Folder(?:AliasType|Icon(?:Resource)?)|IconVariant))|uration(?:Forever|Immediate|Mi(?:crosecond|llisecond))|ynamic(?:RangeControlMode_(?:Heavy|Light|None)|sProcessorParam_(?:AttackTime|CompressionAmount|Expansion(?:Ratio|Threshold)|HeadRoom|InputAmplitude|MasterGain|OutputAmplitude|ReleaseTime|Threshold)))|E(?:A(?:CCESErr|DDR(?:INUSEErr|NOTAVAILErr)|GAINErr|LREADYErr)|B(?:AD(?:FErr|MSGErr)|USYErr)|C(?:ANCELErr|ONN(?:ABORTEDErr|RE(?:FUSEDErr|SETErr)))|DE(?:ADLKErr|STADDRREQErr)|EXISTErr|FAULTErr|HOST(?:DOWNErr|UNREACHErr)|I(?:N(?:PROGRESSErr|TRErr|VALErr)|OErr|SCONNErr)|M(?:SGSIZEErr|ailKCItemAttr)|N(?:ET(?:DOWNErr|RESETErr|UNREACHErr)|O(?:BUFSErr|D(?:ATAErr|EVErr)|ENTErr|M(?:EMErr|SGErr)|PROTOOPTErr|RSRCErr|S(?:RErr|TRErr)|T(?:CONNErr|SOCKErr|TYErr))|XIOErr)|OPNOTSUPPErr|P(?:ERMErr|IPEErr|ROTO(?:Err|NOSUPPORTErr|TYPEErr))|RANGEErr|S(?:HUTDOWNErr|OCKTNOSUPPORTErr|RCHErr)|T(?:IME(?:DOUTErr|Err)|OOMANYREFSErr)|UC_(?:CN_(?:BasicVariant|DOSVariant)|KR_(?:BasicVariant|DOSVariant))|WOULDBLOCKErr|dit(?:TextDialogItem|orsFolderType)|jectMediaIcon|n(?:crypt(?:KCItemAttr|Password)|d(?:CharCode|DateKCItemAttr|Of(?:Sentence|Word))|gravedTextSelector|ter(?:CharCode|Idle|Run|Standby))|scapeCharCode|ve(?:nt(?:A(?:ccessible(?:Get(?:All(?:A(?:ctionNames|ttributeNames)|ParameterizedAttributeNames)|ChildAtPoint|FocusedChild|NamedA(?:ctionDescription|ttribute))|IsNamedAttributeSettable|PerformNamedAction|SetNamedAttribute)|pp(?:A(?:ctiv(?:ated|eWindowChanged)|vailableWindowBoundsChanged)|Deactivated|F(?:ocus(?:Drawer|MenuBar|Next(?:DocumentWindow|FloatingWindow)|Toolbar)|rontSwitched)|GetDockTileMenu|Hidden|IsEventInInstantMouser|Launch(?:Notification|ed)|Quit|S(?:hown|ystemUIModeChanged)|Terminated|UpdateDockTile|earanceScrollBarVariantChanged|leEvent)|ttribute(?:Monitored|None|UserEvent))|C(?:l(?:ass(?:A(?:ccessibility|pp(?:earance|l(?:eEvent|ication)))|C(?:lockView|o(?:mmand|ntrol))|D(?:ataBrowser|elegate)|EPPC|Font|Gesture|HI(?:ComboBox|Object)|Ink|Keyboard|M(?:enu|ouse)|S(?:crollable|e(?:archField|rvice)|ystem)|T(?:SMDocumentAccess|ablet|ext(?:Field|Input)|oolbar(?:Item(?:View)?)?)|Volume|Window)|ockDateOrTimeChanged)|o(?:m(?:boBoxListItemSelected|mand(?:Process|UpdateStatus))|ntrol(?:A(?:ctivate|ddedSubControl|pplyTextColor)|BoundsChanged|C(?:lick|ontextualMenuClick)|D(?:eactivate|ispose|ra(?:g(?:Enter|Leave|Receive|Within)|w))|EnabledStateChanged|FocusPartChanged|G(?:et(?:A(?:ctionProcPart|utoToggleValue)|ClickActivation|Data|F(?:ocusPart|rameMetrics)|IndicatorDragConstraint|NextFocusCandidate|OptimalBounds|Part(?:Bounds|Region)|S(?:crollToHereStartPoint|izeConstraints|ubviewForMouseEvent))|hostingFinished)|Hi(?:liteChanged|t(?:Test)?)|In(?:dicatorMoved|itialize|terceptSubviewClick|validateForSizeChange)|LayoutInfoChanged|O(?:ptimalBoundsChanged|wningWindowChanged)|RemovingSubControl|S(?:et(?:Cursor|Data|FocusPart)|imulateHit)|T(?:itleChanged|rack(?:ingAreaE(?:ntered|xited))?)|V(?:alueFieldChanged|isibilityChanged))))|D(?:ataBrowserDrawCustomItem|elegate(?:Get(?:GroupClasses|TargetClasses)|I(?:nstalled|sGroup)|Removed))|Font(?:PanelClosed|Selection)|Ge(?:sture(?:Ended|Magnify|Rotate|S(?:tarted|wipe))|tSelectedText)|H(?:IObject(?:C(?:onstruct|reatedFromArchive)|Destruct|Encode|GetInitParameters|I(?:nitialize|sEqual)|PrintDebugInfo)|ighLevelEvent|otKey(?:Exclusive|NoOptions|Pressed|Released))|Ink(?:Gesture|Point|Text)|KeyModifier(?:Fn(?:Bit|Mask)|NumLock(?:Bit|Mask))|L(?:eaveInQueue|oopIdleTimer(?:Idling|St(?:arted|opped)))|M(?:enu(?:B(?:ar(?:Hidden|Shown)|e(?:comeScrollable|ginTracking))|C(?:alculateSize|easeToBeScrollable|hangeTrackingMode|losed|reateFrameView)|D(?:ispose|rawItem(?:Content)?)|En(?:ableItems|dTracking)|GetFrameBounds|M(?:atchKey|easureItem(?:Height|Width))|Opening|Populate|TargetItem)|ouse(?:Button(?:Primary|Secondary|Tertiary)|D(?:own|ragged)|E(?:ntered|xited)|Moved|Scroll|Up|Wheel(?:Axis(?:X|Y)|Moved)))|OffsetToPos|P(?:aram(?:A(?:EEvent(?:Class|ID)|TSUFont(?:ID|Size)|ccessib(?:ilityEventQueued|le(?:A(?:ction(?:Description|Name(?:s)?)|ttribute(?:Name(?:s)?|Parameter|Settable|Value))|Child|Object))|fterDelegates|ppleEvent(?:Reply)?|ttributes|vailableBounds)|B(?:eforeDelegates|ounds)|C(?:G(?:ContextRef|ImageRef)|TFontDescriptor|andidateText|lick(?:Activation|Count)|o(?:mboBoxListSelectedItemIndex|ntrol(?:Action|C(?:lickActivationResult|urrent(?:OwningWindow|Part))|D(?:ata(?:Buffer(?:Size)?|Tag)|raw(?:Depth|Engraved|InColor))|F(?:eatures|ocusEverything|rameMetrics)|Hit|I(?:n(?:dicator(?:DragConstraint|Offset|Region)|valRgn)|sGhosting)|Message|O(?:ptimalB(?:aselineOffset|ounds)|riginalOwningWindow)|P(?:ar(?:am|t(?:AutoRepeats|Bounds)?)|re(?:fersShape|viousPart))|Re(?:f|gion|sult)|Sub(?:Control|view)|Value|WouldAcceptDrop))|urrent(?:Bounds|Dock(?:Device|Rect)|MenuTrackingMode|Window))|D(?:ataBrowser(?:Item(?:ID|State)|PropertyID)|e(?:codingForEditor|legate(?:Group(?:Classes|Parameters)|Target(?:Classes)?)|vice(?:Color|Depth))|i(?:ctionary|mensions|rect(?:Object|ionInverted)|splay(?:ChangeFlags|Device))|ragRef)|E(?:nable(?:MenuForKeyEvent|d)|ventRef)|F(?:MFont(?:Family|S(?:ize|tyle))|ontColor)|G(?:Device|rafPort)|HI(?:Archive|Command|ObjectInstance|ViewTrackingArea)|I(?:mageSize|n(?:dex|it(?:Collection|Parameters)|k(?:Gesture(?:Bounds|Hotspot|Kind)|KeyboardShortcut|TextRef))|sInInstantMouser)|Key(?:Code|M(?:acCharCodes|odifiers)|Unicodes|boardType)|L(?:aunch(?:Err|RefCon)|ineSize)|M(?:a(?:gnificationAmount|ximumSize)|enu(?:Co(?:mmand(?:KeyBounds)?|ntext(?:Height)?)|D(?:i(?:rection|smissed)|rawState)|EventOptions|F(?:irstOpen|rameView)|I(?:conBounds|sPopup|tem(?:Bounds|Height|Index|Type|Width))|MarkBounds|PopupItem|Ref|T(?:extB(?:aseline|ounds)|ype)|Virtual(?:Bottom|Top))|inimumSize|o(?:dal(?:ClickResult|Window)|use(?:Button|Chord|Delta|Location|TrackingRef|Wheel(?:Axis|Delta|Smooth(?:HorizontalDelta|VerticalDelta))))|utableArray)|Ne(?:w(?:MenuTrackingMode|ScrollBarVariant)|xtControl)|Origin(?:alBounds)?|P(?:a(?:rentMenu(?:Item)?|steboardRef)|ost(?:Options|Target)|r(?:evious(?:Bounds|Dock(?:Device|Rect)|Window)|ocessID))|R(?:e(?:ason|placementText|sult)|gnHandle|otationAmount)|S(?:crapRef|ervice(?:CopyTypes|MessageName|PasteTypes|UserData)|hape|tartControl|wipeDirection|ystemUI(?:Mode|Options))|T(?:SM(?:DocAccess(?:BaselineDelta|CharacterCount|EffectiveRange|L(?:ineBounds|ockCount)|Re(?:ply(?:ATS(?:Font|UGlyphSelector)|C(?:T(?:FontRef|GlyphInfoRef)|haracter(?:Range|sPtr))|FontSize)|questedCharacterAttributes)|Send(?:C(?:haracter(?:Index|Range|sPtr)|omponentInstance)|RefCon))|Send(?:ComponentInstance|RefCon))|ablet(?:EventType|P(?:oint(?:Rec|erRec)|roximityRec))|ext(?:Input(?:GlyphInfoArray|Reply(?:A(?:TSFont|ttributedString)|CTFontRef|F(?:MFont|ont)|GlyphInfoArray|L(?:eadingEdge|ine(?:Ascent|Height))|MacEncoding|Point(?:Size)?|RegionClass|S(?:LRec|howHide)|Text(?:Angle|Offset)?)|Send(?:AttributedString|C(?:lauseRng|omponentInstance|urrentPoint)|DraggingMode|FixLen|GlyphInfoArray|HiliteRng|KeyboardEvent|LeadingEdge|MouseEvent|PinRng|Re(?:fCon|placeRange)|S(?:LRec|howHide)|Text(?:Offset|Service(?:Encoding|MacEncoding))?|UpdateRng))|Length|Selection)|oolbar(?:Display(?:Mode|Size)|Item(?:ConfigData|Identifier)?)?|ransactionID)|U(?:nconfirmed(?:Range|Text)|serData)|View(?:AttributesDictionary|Size)|Window(?:ContentBounds|D(?:efPart|ragHiliteFlag)|Features|GrowRect|Mo(?:d(?:ality|ifiedFlag)|useLocation)|P(?:artCode|roxy(?:GWorldPtr|ImageRgn|OutlineRgn))|Re(?:f|gionCode)|StateChangedFlags|T(?:itle(?:FullWidth|TextWidth)|ransition(?:Action|Effect))))|osToOffset|r(?:iority(?:High|Low|Standard)|ocessCommand))|QueueOptionsNone|R(?:awKey(?:Down|ModifiersChanged|Repeat|Up)|emoveFromQueue)|S(?:crollable(?:GetInfo|InfoChanged|ScrollTo)|e(?:archField(?:CancelClicked|SearchClicked)|rvice(?:Copy|GetTypes|P(?:aste|erform)))|howHideBottomWindow|ystem(?:Display(?:Reconfigured|sA(?:sleep|wake))|TimeDateChanged|UserSession(?:Activated|Deactivated)))|T(?:SMDocumentAccess(?:Get(?:Characters(?:Ptr(?:ForLargestBuffer)?)?|F(?:irstRectForRange|ont)|GlyphInfo|Length|SelectedRange)|LockDocument|UnlockDocument)|a(?:bletP(?:oint(?:er)?|roximity)|rget(?:DontPropagate|SendToAllHandlers))|ext(?:Accepted|DidChange|Input(?:FilterText|GetSelectedText|IsMouseEventInInlineInputArea|OffsetToPos|PosToOffset|ShowHideBottomWindow|U(?:nicode(?:ForKeyEvent|Text)|pdateActiveInputArea))|ShouldChangeInRange)|oolbar(?:BeginMultiChange|CreateItem(?:FromDrag|WithIdentifier)|Display(?:ModeChanged|SizeChanged)|EndMultiChange|Get(?:AllowedIdentifiers|DefaultIdentifiers|SelectableIdentifiers)|Item(?:A(?:cceptDrop|dded)|C(?:ommandIDChanged|reateCustomView)|EnabledStateChanged|GetPersistentData|HelpTextChanged|ImageChanged|LabelChanged|PerformAction|Removed|SelectedStateChanged|View(?:ConfigFor(?:Mode|Size)|E(?:nterConfigMode|xitConfigMode))|WouldAcceptDrop)|LayoutChanged))|U(?:nicodeForKeyEvent|pdateActiveInputArea)|Volume(?:Mounted|Unmounted)|Window(?:A(?:ctivated|ttributesChanged)|BoundsChang(?:ed|ing)|C(?:lose(?:All|d)?|o(?:l(?:laps(?:e(?:All|d)?|ing)|orSpaceChanged)|n(?:strain|textualMenuSelect))|ursorChange)|D(?:e(?:activated|f(?:D(?:ispose|ra(?:gHilite|w(?:Frame|GrowBox|Part)))|Get(?:GrowImageRegion|Region)|HitTest|Init|M(?:easureTitle|odified)|S(?:etupProxyDragImage|tateChanged)))|ispose|ra(?:g(?:Completed|Hilite|Started)|w(?:Frame|GrowBox|Part|er(?:Clos(?:ed|ing)|Open(?:ed|ing)))))|Expand(?:All|ed|ing)?|F(?:ocus(?:Acquired|Content|Drawer|Lost|Re(?:linquish|stored)|Toolbar)|ullScreenE(?:nter(?:Completed|Started)|xit(?:Completed|Started)))|Get(?:Click(?:Activation|Modality)|DockTileMenu|FullScreenContentSize|GrowImageRegion|IdealS(?:ize|tandardState)|M(?:aximumSize|inimumSize)|Region)|H(?:andle(?:Activate|Deactivate)|i(?:d(?:den|ing)|tTest))|Init|M(?:easureTitle|odified)|P(?:a(?:int|thSelect)|roxy(?:BeginDrag|EndDrag))|Res(?:ize(?:Completed|Started)|tore(?:FromDock|dAfterRelaunch))|S(?:etupProxyDragImage|h(?:eet(?:Clos(?:ed|ing)|Open(?:ed|ing))|ow(?:ing|n))|tateChanged)|T(?:itleChanged|oolbarSwitchMode|ransition(?:Completed|Started))|UpdateDockTile|Zoom(?:All|ed)?))|ryKCEventMask)|x(?:actMatchThread|cludedMemoryException|itIdle|p(?:ertCharactersSelector|o(?:nentsO(?:ffSelector|nSelector)|rtedFolderAliasType))|t(?:AudioFile(?:Error_(?:AsyncWrite(?:BufferOverflow|TooLarge)|Invalid(?:ChannelMap|DataFormat|OperationOrder|Property(?:Size)?|Seek)|MaxPacketSizeUnknown|NonPCMClientFormat)|Property_(?:Audio(?:Converter|File)|C(?:lient(?:ChannelLayout|DataFormat|MaxPacketSize)|o(?:decManufacturer|nverterConfig))|File(?:ChannelLayout|DataFormat|LengthFrames|MaxPacketSize)|IOBuffer(?:SizeBytes)?|PacketTable))|en(?:dedFlag(?:Has(?:CustomBadge|RoutingInfo)|ObjectIsBusy|sAreInvalid)|sion(?:DisabledFolderType|Folder(?:AliasType|Type)|s(?:DisabledFolderIcon|FolderIcon(?:Resource)?))))))|F(?:A(?:AttachCommand|EditCommand|FileParam|IndexParam|RemoveCommand|S(?:erverApp|uiteCode))|BC(?:a(?:ccess(?:Canceled|orStoreFailed)|ddDocFailed|llocFailed|nalysisNotAvailable)|bad(?:IndexFile(?:Version)?|Param|SearchSession)|com(?:mitFailed|pactionFailed)|deletionFailed|f(?:ileNotIndexed|lushFailed)|i(?:llegalSessionChange|ndex(?:CreationFailed|DiskIOFailed|FileDestroyed|Not(?:Available|Found)|ing(?:Canceled|Failed)))|m(?:ergingFailed|oveFailed)|no(?:IndexesFound|S(?:earchSession|uchHit))|s(?:earchFailed|omeFilesNotIndexed|ummarizationCanceled)|tokenizationFailed|v(?:TwinExceptionErr|alidationFailed))|M(?:CurrentFilterFormat|Font(?:C(?:allbackFilterSelector|ontainer(?:AccessErr|FilterSelector))|DirectoryFilterSelector|F(?:amilyCallbackFilterSelector|ileRefFilterSelector)|T(?:ableAccessErr|echnologyFilterSelector))|GenerationFilterSelector|I(?:nvalidFont(?:Err|FamilyErr)|teration(?:Completed|ScopeModifiedErr))|PostScriptFontTechnology|TrueTypeFontTechnology)|N(?:DirectoryModifiedMessage|No(?:ImplicitAllSubscription|tifyInBackground)|S(?:Bad(?:FlattenedSizeErr|ProfileVersionErr|ReferenceVersionErr)|DuplicateReferenceErr|In(?:sufficientDataErr|valid(?:ProfileErr|ReferenceErr))|MismatchErr|NameNotFoundErr))|PUNotNeeded|S(?:Al(?:iasInfo(?:F(?:SInfo|inderInfo)|I(?:Ds|sDirectory)|None|TargetCreateDate|Volume(?:CreateDate|Flags))|lo(?:c(?:AllOrNothingMask|ContiguousMask|DefaultFlags|NoRoundUpMask|ReservedMask)|wConcurrentAsyncIO(?:Bit|Mask)))|CatInfo(?:A(?:ccessDate|llDates|ttrMod)|BackupDate|C(?:ontentMod|reateDate)|DataSizes|F(?:SFileSecurityRef|inder(?:Info|XInfo))|GettableInfo|No(?:de(?:Flags|ID)|ne)|P(?:arentDirID|ermissions)|R(?:eserved|srcSizes)|S(?:et(?:Ownership|tableInfo)|haringFlags)|TextEncoding|User(?:Access|Privs)|V(?:alence|olume))|E(?:jectVolumeForceEject|ventStream(?:CreateFlag(?:FileEvents|IgnoreSelf|No(?:Defer|ne)|UseCFTypes|WatchRoot)|Event(?:Flag(?:EventIdsWrapped|HistoryDone|Item(?:C(?:hangeOwner|reated)|FinderInfoMod|I(?:nodeMetaMod|s(?:Dir|File|Symlink))|Modified|Re(?:moved|named)|XattrMod)|KernelDropped|M(?:ount|ustScanSubDirs)|None|RootChanged|U(?:nmount|serDropped))|IdSinceNow)))|F(?:ileOperation(?:D(?:efaultOptions|oNotMoveAcrossVolumes)|Overwrite|Skip(?:Preflight|SourcePermissionErrors))|orceRead(?:Bit|Mask))|I(?:nvalidVolumeRefNum|terate(?:Delete|Flat|Reserved|Subtree))|KMountVersion|MountServer(?:M(?:arkDoNotDisplay|ount(?:OnMountDir|WithoutNotification))|SuppressConnectionUI)|N(?:ewLine(?:Bit|CharMask|Mask)|o(?:Cache(?:Bit|Mask)|de(?:CopyProtect(?:Bit|Mask)|DataOpen(?:Bit|Mask)|ForkOpen(?:Bit|Mask)|HardLink(?:Bit|Mask)|I(?:nShared(?:Bit|Mask)|s(?:Directory(?:Bit|Mask)|Mounted(?:Bit|Mask)|SharePoint(?:Bit|Mask)))|Locked(?:Bit|Mask)|ResOpen(?:Bit|Mask))))|OperationStage(?:Complete|Preflighting|Running|Undefined)|P(?:athMakeRefD(?:efaultOptions|oNotFollowLeafSymlink)|leaseCache(?:Bit|Mask))|R(?:dVerify(?:Bit|Mask)|eplaceObject(?:D(?:efaultOptions|oNotCheckObjectWriteAccess)|PreservePermissionInfo|Replace(?:Metadata|PermissionInfo)|SaveOriginalAsABackup))|UnmountVolumeForceUnmount|Vol(?:Flag(?:DefaultVolume(?:Bit|Mask)|FilesOpen(?:Bit|Mask)|HardwareLocked(?:Bit|Mask)|JournalingActive(?:Bit|Mask)|SoftwareLocked(?:Bit|Mask))|Info(?:B(?:ackupDate|locks)|C(?:heckedDate|reateDate)|D(?:ataClump|irCount|riveInfo)|F(?:SInfo|i(?:leCount|nderInfo)|lags)|GettableInfo|ModDate|N(?:ext(?:Alloc|ID)|one)|RsrcClump|S(?:ettableInfo|izes))))|TPServerIcon|avorite(?:ItemsIcon|sFolder(?:Icon|Type))|e(?:male|tchReference)|i(?:l(?:eSystemSupportFolderType|lScreen)|nd(?:ByContent(?:FolderType|IndexesFolderType|PluginsFolderType)|SupportFolderType|erIcon)|rst(?:FailKCStopOn|IOKitNotificationType|MagicBusyFiletype|PassKCStopOn)|tToScreen)|l(?:avorType(?:Clipping(?:Filename|Name)|DragToTrashOnly|FinderNoTrackingBehavior|UnicodeClipping(?:Filename|Name))|euronsSelector|o(?:ating(?:PointException|Window(?:Class|Definition))|ppyIconResource))|o(?:lder(?:Action(?:Code|sFolderType)|C(?:losedEvent|reated(?:AdminPrivs(?:Bit)?|Invisible(?:Bit)?|NameLocked(?:Bit)?))|I(?:n(?:LocalOrRemoteUserFolder|RemoteUserFolderIfAvailable(?:Bit)?|UserFolder(?:Bit)?)|tems(?:AddedEvent|RemovedEvent))|M(?:anager(?:FolderInMacOS9FolderIfMacOSXIsInstalled(?:Bit|Mask)|LastDomain|N(?:ewlyCreatedFolder(?:IsLocalizedBit|ShouldHaveDotLocalizedCreatedWithinMask)|otCreatedOnRemoteVolumes(?:Bit|Mask)))|ustStayOnSameVolume(?:Bit)?)|NeverMatchedInIdentifyFolder(?:Bit)?|OpenedEvent|TrackedByAlias(?:Bit)?|WindowMovedEvent)|nt(?:A(?:lbanianLanguage|mharic(?:Language|Script)|r(?:abic(?:Language|Script)|menian(?:Language|Script))|ssameseLanguage|ymaraLanguage|zerbaijan(?:ArLanguage|iLanguage))|B(?:asqueLanguage|engali(?:Language|Script)|u(?:lgarianLanguage|rmese(?:Language|Script))|yelorussianLanguage)|C(?:atalanLanguage|h(?:ewaLanguage|ineseScript)|o(?:llectionsFolderType|pyrightName)|roatianLanguage|ustom(?:16BitScript|8(?:16BitScript|BitScript)|Platform)|yrillicScript|zechLanguage)|D(?:anishLanguage|e(?:s(?:criptionName|igner(?:Name|URLName))|vanagariScript)|utchLanguage|zongkhaLanguage)|E(?:astEuropeanRomanScript|nglishLanguage|s(?:perantoLanguage|tonianLanguage)|thiopicScript|xtendedArabicScript)|F(?:a(?:eroeseLanguage|milyName|rsiLanguage)|innishLanguage|lemishLanguage|renchLanguage|ullName)|G(?:allaLanguage|e(?:ezScript|orgian(?:Language|Script)|rmanLanguage)|reek(?:Language|Script)|u(?:araniLanguage|jarati(?:Language|Script)|rmukhiScript))|H(?:ebrew(?:Language|Script)|indiLanguage|ungarianLanguage)|I(?:SO10646_1993Semantics|celandicLanguage|ndonesianLanguage|rishLanguage|talianLanguage)|Ja(?:panese(?:Language|Script)|vaneseRomLanguage)|K(?:a(?:nnada(?:Language|Script)|shmiriLanguage|zakhLanguage)|hmer(?:Language|Script)|irghizLanguage|orean(?:Language|Script)|urdishLanguage)|L(?:a(?:o(?:Language|tianScript)|ppishLanguage|stReservedName|t(?:inLanguage|vianLanguage))|ettishLanguage|i(?:cense(?:DescriptionName|InfoURLName)|thuanianLanguage))|M(?:a(?:c(?:CompatibleFullName|edonianLanguage|intoshPlatform)|l(?:a(?:gasyLanguage|y(?:ArabicLanguage|RomanLanguage|alam(?:Language|Script)))|teseLanguage)|nufacturerName|rathiLanguage)|icrosoft(?:Platform|S(?:tandardScript|ymbolScript)|UCS4Script)|o(?:ldavianLanguage|ngolian(?:CyrLanguage|Language|Script)))|N(?:epaliLanguage|o(?:Language(?:Code)?|Name(?:Code)?|Platform(?:Code)?|Script(?:Code)?|rwegianLanguage))|Or(?:iya(?:Language|Script)|omoLanguage)|P(?:ashtoLanguage|ersianLanguage|o(?:lishLanguage|rtugueseLanguage|st(?:ScriptCIDName|scriptName))|referred(?:FamilyName|SubfamilyName)|unjabiLanguage)|QuechuaLanguage|R(?:SymbolScript|eservedPlatform|oman(?:Script|ianLanguage)|u(?:andaLanguage|ndiLanguage|ssian(?:Language)?))|S(?:a(?:amiskLanguage|mpleTextName|nskritLanguage)|e(?:lection(?:ATSUIType|CoreTextType|QD(?:StyleVersionZero|Type))|rbianLanguage)|i(?:mp(?:ChineseLanguage|leChineseScript)|n(?:dhi(?:Language|Script)|halese(?:Language|Script)))|l(?:avicScript|ov(?:akLanguage|enianLanguage))|omaliLanguage|panishLanguage|tyleName|u(?:itcaseIcon|ndaneseRomLanguage)|w(?:ahiliLanguage|edishLanguage))|T(?:a(?:galogLanguage|jikiLanguage|mil(?:Language|Script)|tarLanguage)|elugu(?:Language|Script)|hai(?:Language|Script)|i(?:betan(?:Language|Script)|grinyaLanguage)|rad(?:ChineseLanguage|emarkName|itionalChineseScript)|urk(?:ishLanguage|menLanguage))|U(?:ighurLanguage|krainianLanguage|ni(?:code(?:DefaultSemantics|Platform|V(?:1_1Semantics|2_0(?:BMPOnlySemantics|FullCoverageSemantics)|4_0VariationSequenceSemantics)|_FullRepertoire)|nterpretedScript|queName)|rduLanguage|zbekLanguage)|V(?:e(?:ndorURLName|rsionName)|ietnamese(?:Language|Script))|WelshLanguage|YiddishLanguage|sFolder(?:Icon(?:Resource)?|Type))|r(?:kInfoFlags(?:FileLocked(?:Bit|Mask)|LargeFile(?:Bit|Mask)|Modified(?:Bit|Mask)|OwnClump(?:Bit|Mask)|Resource(?:Bit|Mask)|SharedWrite(?:Bit|Mask)|Write(?:Bit|Locked(?:Bit|Mask)|Mask))|m(?:FeedCharCode|InterrobangO(?:ffSelector|nSelector))|wardArrowIcon)|urByteCode)|ra(?:ctionsType|gment(?:IsPrepared|NeedsPreparing)|me(?:buffer(?:DisableAltivecAccess|Supports(?:CopybackCache|GammaCorrection|WritethruCache))|worksFolderType))|u(?:ll(?:TrashIcon(?:Resource)?|Width(?:CJKRomanSelector|IdeographsSelector|KanaSelector))|nctionKeyCharCode))|G(?:SSSelect(?:Ge(?:nericToRealID|t(?:DefaultScriptingComponent|ScriptingComponent(?:FromStored)?))|OutOfRange|RealToGenericID|SetDefaultScriptingComponent)|UARD_EXC_(?:DE(?:ALLOC_GAP|STROY)|I(?:MMOVABLE|N(?:CORRECT_GUARD|VALID_(?:ARGUMENT|NAME|RIGHT|VALUE)))|KERN_(?:FAILURE|NO_SPACE|RESOURCE)|MOD_REFS|R(?:CV_(?:GUARDED_DESC|INVALID_NAME)|IGHT_EXISTS)|S(?:E(?:ND_INVALID_(?:R(?:EPLY|IGHT)|VOUCHER)|T_CONTEXT)|TRICT_REPLY)|UNGUARDED)|e(?:n(?:EditorsFolderType|er(?:alFailureErr|ic(?:ApplicationIcon(?:Resource)?|C(?:DROMIcon(?:Resource)?|o(?:mponent(?:Icon|Version)|ntrol(?:PanelIcon|StripModuleIcon)))|D(?:eskAccessoryIcon(?:Resource)?|ocumentIcon(?:Resource)?)|E(?:ditionFileIcon(?:Resource)?|xtensionIcon(?:Resource)?)|F(?:ileServerIcon(?:Resource)?|loppyIcon|o(?:lderIcon(?:Resource)?|nt(?:Icon|ScalerIcon)))|HardDiskIcon(?:Resource)?|IDiskIcon|KCItemAttr|MoverObjectIcon(?:Resource)?|NetworkIcon|P(?:CCardIcon|asswordKCItemClass|referencesIcon(?:Resource)?)|QueryDocumentIcon(?:Resource)?|R(?:AMDiskIcon(?:Resource)?|emovableMediaIcon)|S(?:haredLibaryIcon|tationeryIcon(?:Resource)?|uitcaseIcon(?:Resource)?)|URLIcon|W(?:ORMIcon|indowIcon))))|t(?:AE(?:TE|UT)|DebugOption|Power(?:Info|Level)|SelectedText|WakeOnNetInfo))|lyphCollection(?:Adobe(?:CNS1|GB1|Japan(?:1|2)|Korea1)|GID|Unspecified)|r(?:aphicEQParam_NumberOfBands|idIcon|oup(?:I(?:D2Name|con)|Name2ID))|uestUserIcon)|H(?:ALOutputParam_Volume|FS(?:A(?:llocationFileID|ttribute(?:DataFileID|sFileID)|utoCandidate(?:Bit|Mask))|B(?:adBlockFileID|inaryCompare|o(?:gusExtentFileID|otVolumeInconsistent(?:Bit|Mask)))|C(?:a(?:seFolding|talog(?:FileID|KeyM(?:aximumLength|inimumLength)|NodeIDsReused(?:Bit|Mask)))|ontentProtection(?:Bit|Mask))|DoNotFastDevPin(?:Bit|Mask)|Extent(?:Density|KeyMaximumLength|sFileID)|F(?:astDev(?:Candidate(?:Bit|Mask)|Pinned(?:Bit|Mask))|i(?:le(?:Locked(?:Bit|Mask)|Record|ThreadRecord)|rstUserCatalogNodeID)|older(?:Record|ThreadRecord))|Has(?:Attributes(?:Bit|Mask)|ChildLink(?:Bit|Mask)|DateAdded(?:Bit|Mask)|FolderCount(?:Bit|Mask)|LinkChain(?:Bit|Mask)|Security(?:Bit|Mask))|JMountVersion|M(?:DBAttributesMask|ax(?:AttrNameLen|FileNameChars|VolumeNameChars))|Plus(?:Attr(?:Extents|ForkData|InlineData|MinNodeSize)|C(?:atalog(?:KeyM(?:aximumLength|inimumLength)|MinNodeSize)|reator)|Extent(?:Density|KeyMaximumLength|MinNodeSize)|F(?:ile(?:Record|ThreadRecord)|older(?:Record|ThreadRecord))|M(?:axFileNameChars|ountVersion)|SigWord|Version)|R(?:epairCatalogFileID|oot(?:FolderID|ParentID))|S(?:igWord|tartupFileID)|ThreadExists(?:Bit|Mask)|UnusedNode(?:Fix(?:Bit|Mask)|sFixDate)|Volume(?:HardwareLock(?:Bit|Mask)|Inconsistent(?:Bit|Mask)|Journaled(?:Bit|Mask)|NoCacheRequired(?:Bit|Mask)|S(?:oftwareLock(?:Bit|Mask)|paredBlocks(?:Bit|Mask))|Unmounted(?:Bit|Mask))|X(?:SigWord|Version))|I(?:ArchiveDecod(?:eSuperclassForUnregisteredObjects|ingForEditor)|C(?:lassOptionSingleton|o(?:m(?:boBox(?:Auto(?:CompletionAttribute|DisclosureAttribute|S(?:izeListAttribute|ortAttribute))|DisclosurePart|EditTextPart|List(?:Pixel(?:HeightTag|WidthTag)|Tag)|N(?:oAttributes|umVisibleItemsTag)|StandardAttributes)|mand(?:A(?:bout|ppHelp|rrangeInFront)|BringAllToFront|C(?:ancel|h(?:angeSpelling|eckSpelling(?:AsYouType)?)|l(?:ear|ose(?:All|File)?)|opy|u(?:stomizeToolbar|t)|ycleToolbarMode(?:Larger|Smaller))|From(?:Control|Menu|Window)|Hide(?:Others|Toolbar)?|IgnoreSpelling|LearnWord|M(?:aximize(?:All|Window)|inimize(?:All|Window))|New|O(?:K|pen|ther)|P(?:a(?:geSetup|ste)|r(?:eferences|int))|Quit(?:And(?:DiscardWindows|KeepWindows))?|R(?:e(?:do|vert)|otate(?:FloatingWindows(?:Backward|Forward)|Windows(?:Backward|Forward)))|S(?:ave(?:As)?|elect(?:All|Window)|how(?:All|CharacterPalette|HideFontPanel|SpellingPanel|Toolbar)|tartDictation)|Toggle(?:AllToolbars|FullScreen|Toolbar)|Undo|WindowList(?:Separator|Terminator)|ZoomWindow))|ordSpace(?:72DPIGlobal|ScreenPixel|View|Window)))|D(?:B(?:a(?:d(?:Log(?:PhysValuesErr|icalM(?:aximumErr|inimumErr))|ParameterErr)|seError)|ufferTooSmallErr)|DeviceNotReady|EndOfDescriptorErr|In(?:compatibleReportErr|v(?:alid(?:PreparsedDataErr|R(?:angePageErr|eport(?:LengthErr|TypeErr)))|erted(?:LogicalRangeErr|PhysicalRangeErr|UsageRangeErr)))|N(?:ot(?:EnoughMemoryErr|ValueArrayErr)|ull(?:PointerErr|StateErr))|Report(?:CountZeroErr|IDZeroErr|SizeZeroErr)|Success|U(?:nmatched(?:DesignatorRangeErr|StringRangeErr|UsageRangeErr)|sage(?:NotFoundErr|PageZeroErr))|V(?:alueOutOfRangeErr|ersionIncompatibleErr)|elegate(?:A(?:fter|ll)|Before))|HotKeyModeAll(?:Disabled(?:ExceptUniversalAccess)?|Enabled)|ImageView(?:AutoTransform(?:None|OnD(?:eactivate|isable))|ImageTag)|Layout(?:Bind(?:Bottom|Left|M(?:ax|in)|None|Right|Top)|InfoVersionZero|Position(?:Bottom|Center|Left|M(?:ax|in)|None|Right|Top)|ScaleAbsolute)|M(?:enu(?:AppendItem|CenterDirection|DismissedBy(?:A(?:ctivationChange|ppSwitch)|CancelMenuTracking|FocusChange|KeyEvent|Mouse(?:Down|Up)|Selection|Timeout|UserCancel)|LeftDirection|RightDirection)|odalClick(?:A(?:llowEvent|nnounce)|IsModal|RaiseWindow))|S(?:crollView(?:Options(?:AllowGrow|DisableSmoothScrolling|FillGrowArea|HorizScroll|VertScroll)|Page(?:Down|Left|Right|Up)|ScrollTo(?:Bottom|Left|Right|Top)|ValidOptions)|e(?:archField(?:Attributes(?:Cancel|SearchIcon)|NoAttributes)|gment(?:Behavior(?:Momentary|Radio|Sticky|Toggles)|NoAttributes|SendCmdToUserFocus|edViewKind))|hape(?:Enumerate(?:Init|Rect|Terminate)|ParseFrom(?:Bottom(?:Right)?|Left|Right|Top(?:Left)?)))|T(?:heme(?:F(?:ocusRing(?:Above|Below|Only)|rame(?:ListBox|TextField(?:Round(?:Mini|Small)?|Square)))|Gro(?:upBoxKind(?:Primary(?:Opaque)?|Secondary(?:Opaque)?)|wBox(?:KindNo(?:ne|rmal)|Size(?:Normal|Small)))|HeaderKind(?:List|Window)|Menu(?:DrawInfoVersion(?:One|Zero)|TitleDrawCondensed)|Orientation(?:Inverted|Normal)|S(?:egment(?:Adornment(?:Focus|LeadingSeparator|None|TrailingSeparator)|Kind(?:Inset|Normal|Textured)|Position(?:First|Last|Middle|Only)|Size(?:Mini|Normal|Small))|plitterAdornment(?:Metal|None))|T(?:ab(?:Adornment(?:Focus|LeadingSeparator|None|TrailingSeparator)|KindNormal|P(?:aneAdornmentNormal|osition(?:First|Last|Middle|Only))|Size(?:Mini|Normal|Small))|ext(?:BoxOption(?:DontClip|Engraved|None|StronglyVertical)|HorizontalFlush(?:Center|Default|Left|Right)|InfoVersion(?:One|Zero)|Truncation(?:Default|End|Middle|None)|VerticalFlush(?:Bottom|Center|Default|Top))))|oolbar(?:AutoSavesConfig|CommandPressAction|Display(?:Mode(?:Default|Icon(?:AndLabel|Only)|LabelOnly)|Size(?:Default|Normal|Small))|I(?:sConfigurable|tem(?:A(?:llowDuplicates|nchoredLeft)|CantBeRemoved|Disabled|IsSeparator|LabelDisabled|MutableAttrs|NoAttributes|Se(?:lected|ndCmdToUserFocus)|ValidAttrs))|NoAttributes|V(?:alidAttrs|iewDrawBackgroundTag))|ransform(?:Disabled|None|Selected))|View(?:A(?:llowsSubviews|ttribute(?:IsFieldEditor|SendCommandToUserFocus)|utoToggles)|C(?:lickableMetaPart|ontent(?:AlertIconType|CGImageRef|I(?:con(?:Ref|SuiteRef|TypeAndCreator)|mage(?:File|Resource))|MetaPart|N(?:SImage|one)|TextOnly))|D(?:isabledPart|oesNot(?:Draw|UseSpecialParts))|EntireView|F(?:eature(?:A(?:llowsSubviews|utoToggles)|DoesNot(?:Draw|UseSpecialParts)|GetsFocusOnClick|I(?:dlesWithTimer|gnoresClicks|nvertsUpDownValueMeaning|sOpaque)|Supports(?:Ghosting|LiveFeedback|RadioBehavior))|ocus(?:N(?:extPart|oPart)|OnAnyControl|PrevPart|Traditionally|WithoutWrapping))|GetsFocusOnClick|I(?:dlesWithTimer|gnoresClicks|n(?:activePart|dicatorPart|vertsUpDownValueMeaning)|sOpaque)|KindSignatureApple|NoPart|O(?:ffscreenImageUseWindowBackingResolution|paqueMetaPart)|S(?:endCommandToUserFocus|tructureMetaPart|upports(?:Ghosting|LiveFeedback|RadioBehavior))|ValidFeaturesForPanther|ZOrder(?:Above|Below))|Window(?:B(?:ackingLocation(?:Default|MainMemory|VideoMemory)|ehavior(?:Stationary|Transient)|it(?:A(?:syncDrag|uto(?:Calibration|ViewDragTracking))|C(?:anBeVisibleWithoutLogin|loseBox|o(?:llapseBox|mpositing))|DoesNot(?:Cycle|Hide)|F(?:rameworkScaled|ullScreen(?:Auxiliary|Primary))|Hi(?:deOn(?:FullScreen|Suspend)|ghResolutionCapable)|I(?:gnoreClicks|nWindowMenu)|LiveResize|No(?:Activates|Constrain|Shadow|T(?:exturedContentSeparator|itleBar)|Updates)|OpaqueForEvents|R(?:esizable|oundBottomBarCorners)|S(?:ideTitlebar|tandardHandler)|T(?:extured(?:SquareCorners)?|oolbarButton)|UnifiedTitleAndToolbar|ZoomBox))|CanJoinAllSpaces|D(?:epth(?:32Bit|64Bit|Float|Invalid)|ragPart)|ExposeHidden|IgnoreObscuringWindows|M(?:enu(?:Creator|WindowTag)|oveToActiveSpace)|S(?:caleMode(?:FrameworkScaled|Magnified|Unscaled)|haring(?:None|Read(?:Only|Write)))|Title(?:BarPart|ProxyIconPart)|VisibleInAllSpaces))|M(?:AbsoluteCenterAligned|Bottom(?:LeftCorner|RightCorner|Side)|C(?:FString(?:Content|LocalizedContent)|ontent(?:NotProvided(?:DontPropagate)?|Provided))|D(?:efaultSide|isposeContent)|H(?:elpMenuID|ideTag(?:Fade|Immediately))|I(?:llegalContentForMinimumState|nside(?:Bottom(?:CenterAligned|LeftCorner|RightCorner)|LeftCenterAligned|RightCenterAligned|Top(?:CenterAligned|LeftCorner|RightCorner)))|Left(?:BottomCorner|Side|TopCorner)|M(?:aximumContentIndex|inimumContentIndex)|NoContent|Outside(?:Bottom(?:CenterAligned|LeftAligned|RightAligned|ScriptAligned)|Left(?:BottomAligned|CenterAligned|TopAligned)|Right(?:BottomAligned|CenterAligned|TopAligned)|Top(?:CenterAligned|LeftAligned|RightAligned|ScriptAligned))|PascalStrContent|Right(?:BottomCorner|Side|TopCorner)|S(?:tr(?:ResContent|ingResContent)|upplyContent)|T(?:EHandleContent|extResContent|op(?:LeftCorner|RightCorner|Side)))|TTPServerIcon|a(?:lfWidth(?:CJKRomanSelector|IdeographsSelector|TextSelector)|n(?:dle(?:IsResource(?:Bit|Mask)|Locked(?:Bit|Mask)|Purgeable(?:Bit|Mask))|jaToHangul(?:Alt(?:OneSelector|T(?:hreeSelector|woSelector))|Selector))|rd(?:LinkFileType|wareCursor(?:DescriptorM(?:ajorVersion|inorVersion)|InfoM(?:ajorVersion|inorVersion)))|s(?:B(?:eenInited|undle)|CustomIcon|NoINITs))|e(?:brew(?:FigureSpaceVariant|StandardVariant)|lp(?:CharCode|DialogItem|Folder(?:Icon|Type)|Icon(?:Resource)?|TagEventHandlerTag|WindowClass))|i(?:deDiacriticsSelector|erarchicalFontMenuOption|gh(?:LevelEvent|ShelfParam_(?:CutOffFrequency|Gain))|nt(?:Advanced|Basic|Hidden)|passParam_(?:CutoffFrequency|Resonance)|raganaToKatakanaSelector|storicalLigaturesO(?:ffSelector|nSelector))|o(?:joCharactersSelector|meCharCode|rizontalConstraint)|uge(?:1BitMask|32BitData|4BitData|8Bit(?:Data|Mask))|yphen(?:To(?:EnDashO(?:ffSelector|nSelector)|MinusO(?:ffSelector|nSelector))|sToEmDashO(?:ffSelector|nSelector)))|I(?:BCarbonRuntime(?:CantFind(?:NibFile|Object)|ObjectNotOfRequestedType)|C(?:Attr(?:Locked(?:Bit|Mask)|NoChange|Volatile(?:Bit|Mask))|C(?:omponent(?:InterfaceVersion(?:0|1|2|3|4)?|Version)|reator)|EditPreferenceEvent(?:Class)?|File(?:SpecHeaderSize|Type)|Map(?:Binary(?:Bit|Mask)|DataFork(?:Bit|Mask)|FixedLength|Not(?:Incoming(?:Bit|Mask)|Outgoing(?:Bit|Mask))|Post(?:Bit|Mask)|ResourceFork(?:Bit|Mask))|N(?:ilProfileID|oUserInteraction(?:Bit|Mask)|umVersion)|Services(?:TCP(?:Bit|Mask)|UDP(?:Bit|Mask)))|MJaTypingMethod(?:Kana|Property|Roman)|O(?:A(?:nalogS(?:etupExpected|ignalLevel_(?:07(?:00_0(?:000|300)|14_0286)|1000_0400))|sync(?:C(?:allout(?:Count|FuncIndex|RefconIndex)|ompletionNotificationType)|Reserved(?:Count|Index)))|B(?:itsPerColorComponent(?:1(?:0|2|6)|6|8|NotSupported)|uiltinPanelPowerAttribute)|C(?:LUTPixels|SyncDisable|apturedAttribute|lamshellStateAttribute|o(?:lorimetry(?:AdobeRGB|BT(?:2(?:020|100)|601|709)|DCIP3|N(?:ativeRGB|otSupported)|WGRGB|sRGB|xvYCC)|nnect(?:MethodVarOutputSize|ion(?:BuiltIn|StereoSync))|pyback(?:Cache|InnerCache))|ursorControlAttribute)|D(?:PEvent(?:AutomatedTestRequest|ContentProtection|ForceRetrain|Idle|MCCS|RemoteControlCommandPending|S(?:inkSpecific|tart))|SCBlockPredEnable|e(?:f(?:ault(?:Cache|MemoryType)|erCLUTSetAttribute)|tailedTimingValid)|i(?:gitalSignal|splay(?:ColorMode|Dither(?:All|D(?:efault|isable)|FrameRateControl|RGBShift|Spatial|Temporal|YCbCr4(?:22Shift|44Shift))|ModeID(?:BootProgrammable|ReservedBase)|NeedsCEAUnderscan|PowerState(?:MinUsable|O(?:ff|n))|RGBColorComponentBits(?:1(?:0|2|4|6)|6|8|Unknown)|YCbCr4(?:22ColorComponentBits(?:1(?:0|2|4|6)|6|8|Unknown)|44ColorComponentBits(?:1(?:0|2|4|6)|6|8|Unknown))))|riverPowerAttribute|ynamicRange(?:Dolby(?:NormalMode|TunnelMode)|HDR10|NotSupported|SDR|TraditionalGammaHDR))|F(?:B(?:AVSignalType(?:D(?:P|VI)|HDMI|Unknown|VGA)|B(?:itRate(?:HBR(?:2)?|RBR)|lueGammaScaleAttribute)|C(?:hangedInterruptType|onnectInterruptType)|Display(?:Port(?:InterruptType|LinkChangeInterruptType|TrainingAttribute)|State(?:_(?:AlreadyActive|Mask|PipelineBlack|RestoredProfile))?)|FrameInterruptType|GreenGammaScaleAttribute|H(?:BLInterruptType|D(?:CPLimit_(?:AllowAll|NoHDCP(?:1x|20Type(?:0|1)))|RMetaDataAttribute))|Li(?:mitHDCP(?:Attribute|StateAttribute)|nk(?:Downspread(?:Max|None)|PreEmphasisLevel(?:0|1|2|3)|Scrambler(?:Alternate|Normal)|VoltageLevel(?:0|1|2|3)))|MCCSInterruptType|NS_(?:D(?:i(?:m|splayState(?:Mask|Shift))|oze)|Generation(?:Mask|Shift)|MessageMask|Rendezvous|Sleep|UnDim|Wake)|O(?:fflineInterruptType|nlineInterruptType)|RedGammaScaleAttribute|S(?:erverConnectType|haredConnectType|top|ystemAperture)|UserRequestProbe|V(?:BLInterruptType|ariableRefreshRate)|WakeInterruptType)|ixedCLUTPixels)|GDiagnose(?:ConnectType|GTraceType)|H(?:SyncDisable|ardwareCursorAttribute|ibernatePreview(?:Active|Updates))|In(?:hibitCache|ter(?:estCallout(?:Count|FuncIndex|RefconIndex|ServiceIndex)|lacedCEATiming))|KitNotication(?:MsgSizeMask|Type(?:Mask|SizeAdjShift))|M(?:a(?:p(?:Anywhere|C(?:ache(?:Mask|Shift)|opyback(?:Cache|InnerCache))|DefaultCache|InhibitCache|Overwrite|P(?:osted(?:CombinedReordered|Reordered|Write)|refault)|Re(?:a(?:dOnly|lTimeCache)|ference)|Static|U(?:nique|serOptionsMask)|Write(?:CombineCache|ThruCache))|tchingCallout(?:Count|FuncIndex|RefconIndex)|xPixelBits)|irror(?:Attribute|Default(?:Attribute)?|Forced|HWClipped|Is(?:Mirrored|Primary))|ono(?:DirectPixels|InverseDirectPixels))|N(?:TSCTiming|oSeparateSyncControl)|P(?:ALTiming|ixelEncoding(?:NotSupported|RGB444|YCbCr4(?:2(?:0|2)|44))|o(?:sted(?:CombinedReordered|Reordered|Write)|wer(?:Attribute|StateAttribute)))|R(?:GB(?:DirectPixels|Signed(?:DirectPixels|FloatingPointPixels))|ange(?:BitsPerColorComponent(?:1(?:0|2|6)|6|8|NotSupported)|Colorimetry(?:AdobeRGB|BT(?:2(?:020|100)|601|709)|DCIP3|N(?:ativeRGB|otSupported)|WGRGB|sRGB|xvYCC)|DynamicRange(?:Dolby(?:NormalMode|TunnelMode)|HDR10|NotSupported|SDR|TraditionalGammaHDR)|PixelEncoding(?:NotSupported|RGB444|YCbCr4(?:2(?:0|2)|44))|Supports(?:CompositeSync|InterlacedCEATiming(?:WithConfirm)?|S(?:eparateSyncs|ignal_(?:07(?:00_0(?:000|300)|14_0286)|1000_0400)|yncOnGreen)|VSyncSerration))|e(?:alTimeCache|gistryIterate(?:Parents|Recursively)))|S(?:cal(?:e(?:Can(?:BorderInsetOnly|DownSamplePixels|Rotate|S(?:caleInterlaced|upportInset)|UpSamplePixels)|Invert(?:X|Y)|Rotate(?:0|180|270|90|Flags)|S(?:tretch(?:Only|ToFit)|wapAxes))|ingInfoValid)|ervice(?:InteractionAllowed|M(?:atchedNotificationType|essageNotificationType)|PublishNotificationType|TerminatedNotificationType)|urface(?:Co(?:mponent(?:Name(?:Alpha|Blue|Chroma(?:Blue|Red)|Green|Luma|Red|Unknown)|Range(?:FullRange|Unknown|VideoRange|WideRange)|Type(?:Float|SignedInteger|Un(?:known|signedInteger)))|pyback(?:Cache|InnerCache))|DefaultCache|InhibitCache|Lock(?:AvoidSync|ReadOnly)|Map(?:C(?:acheShift|opyback(?:Cache|InnerCache))|DefaultCache|InhibitCache|Write(?:CombineCache|ThruCache))|Purgeable(?:Empty|KeepCurrent|NonVolatile|Volatile)|Subsampling(?:4(?:11|2(?:0|2))|None|Unknown)|Write(?:CombineCache|ThruCache))|y(?:nc(?:On(?:Blue|Green|Red)|PositivePolarity)|stemPowerAttribute))|T(?:iming(?:ID(?:Apple(?:NTSC_(?:FF(?:conv)?|ST(?:conv)?)|PAL_(?:FF(?:conv)?|ST(?:conv)?)|_(?:0x0_0hz_Offline|1(?:024x768_75hz|152x870_75hz)|5(?:12x384_60hz|60x384_60hz)|640x(?:4(?:00_67hz|80_67hz)|8(?:18_75hz|70_75hz))|832x624_75hz|FixedRateLCD))|FilmRate_48hz|GTF_640x480_120hz|Invalid|S(?:MPTE240M_60hz|ony_1(?:600x1024_76hz|920x1(?:080_(?:60hz|72hz)|200_76hz)))|VESA_(?:1(?:024x768_(?:60hz|7(?:0hz|5hz)|85hz)|152x864_75hz|280x(?:1024_(?:60hz|75hz|85hz)|960_(?:60hz|75hz|85hz))|360x768_60hz|600x1200_(?:6(?:0hz|5hz)|7(?:0hz|5hz)|8(?:0hz|5hz))|792x1344_(?:60hz|75hz)|856x1392_(?:60hz|75hz)|920x1440_(?:60hz|75hz))|640x480_(?:60hz|7(?:2hz|5hz)|85hz)|8(?:00x600_(?:56hz|60hz|7(?:2hz|5hz)|85hz)|48x480_60hz)))|RangeV(?:1|2))|riStateSyncs)|V(?:RAMSaveAttribute|SyncDisable)|W(?:SAA_(?:Accelerated|D(?:efer(?:End|Start)|riverOpen)|From_Accelerated|Hibernate|NonConsoleDevice|Reserved|S(?:leep|tateMask)|T(?:o_Accelerated|ransactional)|Unaccelerated)|indowServerActiveAttribute|rite(?:CombineCache|ThruCache)))|PFileServerIcon|S(?:OLatin(?:1(?:MusicCDVariant|StandardVariant)|Arabic(?:ExplicitOrderVariant|ImplicitOrderVariant|VisualOrderVariant)|Hebrew(?:ExplicitOrderVariant|ImplicitOrderVariant|VisualOrderVariant))|SDownloadsFolderType|p(?:BufferToSmallErr|Device(?:ActiveErr|InactiveErr)|Element(?:InListErr|NotInListErr)|InternalErr|ListBusyErr|System(?:ActiveErr|InactiveErr|ListErr)))|con(?:DialogItem|FamilyType|Services(?:1(?:024PixelDataARGB|28PixelDataARGB|6PixelDataARGB)|256PixelDataARGB|32PixelDataARGB|48PixelDataARGB|512PixelDataARGB|CatalogInfoMask|No(?:BadgeFlag|rmalUsageFlag)|UpdateIfNeededFlag))|d(?:eographic(?:Alt(?:F(?:iveSelector|ourSelector)|OneSelector|T(?:hreeSelector|woSelector)|ernativesType)|SpacingType)|leKCEvent(?:Mask)?)|ll(?:egal(?:ClockValueErr|InstructionException)|uminatedCapsSelector)|mmediate|n(?:DeferredTaskMask|NestedInterruptMask|SecondaryIntHandlerMask|UseErr|VBLTaskMask|dexFilesFolderType|equalityLigaturesO(?:ffSelector|nSelector)|feriorsSelector|itialCaps(?:AndSmallCapsSelector|Selector)|kInputMethodClass|putM(?:anagersFolderType|ethodsFolderType)|s(?:ertHierarchicalMenu|t(?:aller(?:LogsFolderType|ReceiptsFolderType)|ru(?:ctionBreakpointException|mentType_(?:A(?:UPreset|udiofile)|DLSPreset|EXS24|SF2Preset))))|te(?:gerException|rn(?:ation(?:ResourcesIcon|al(?:ResourcesIcon|SymbolsSelector))|et(?:EventClass|Folder(?:Icon|Type)|Location(?:A(?:FP|pple(?:ShareIcon|Talk(?:ZoneIcon)?))|Creator|F(?:TP(?:Icon)?|ile(?:Icon)?)|Generic(?:Icon)?|HTTP(?:Icon)?|Mail(?:Icon)?|N(?:NTP|SL(?:NeighborhoodIcon)?|ewsIcon))|P(?:asswordKCItemClass|lugInFolder(?:Icon|Type))|S(?:earchSitesFolder(?:Icon|Type)|itesFolderType))))|v(?:alid(?:CSClientErr|DeviceNumber|Font(?:Family)?|Generation|RegEntryErr)|ert(?:Highlighting|ed(?:BoxAnnotationSelector|CircleAnnotationSelector|RoundedBoxAnnotationSelector)|ingEncod(?:edPixel|ing(?:Shift)?))|isibleKCItemAttr))|s(?:Alias|Invisible|OnDesk|S(?:hared|tationery)|suer(?:KCItemAttr|URLKCItemAttr))|t(?:alicCJKRomanType|em(?:DisableBit|List)))|J(?:I(?:Journal(?:InFSMask|NeedInitMask|OnOtherDeviceMask)|S(?:19(?:78CharactersSelector|83CharactersSelector|90CharactersSelector)|2004CharactersSelector))|S(?:ClassAttributeNo(?:AutomaticPrototype|ne)|PropertyAttribute(?:Dont(?:Delete|Enum)|None|ReadOnly)|Type(?:Boolean|Nu(?:ll|mber)|Object|String|Undefined|dArrayType(?:ArrayBuffer|Float(?:32Array|64Array)|Int(?:16Array|32Array|8Array)|None|Uint(?:16Array|32Array|8(?:Array|ClampedArray)))))|UST(?:CurrentVersion|KashidaPriority|LetterPriority|NullPriority|Override(?:Limits|Priority|Unlimited)|Priority(?:Count|Mask)|S(?:pacePriority|tandardFormat)|Tag|Unlimited|noGlyphcode|pc(?:ConditionalAddAction|D(?:ecompositionAction|uctilityAction)|Glyph(?:RepeatAddAction|StretchAction)|UnconditionalAddAction))|apanese(?:BasicVariant|PostScript(?:PrintVariant|ScrnVariant)|St(?:andardVariant|dNoVerticalsVariant)|VertAtKuPlusTenVariant))|K(?:C(?:AuthType(?:D(?:PA|efault)|HTTPDigest|MSN|NTLM|RPA)|ProtocolType(?:A(?:FP|ppleTalk)|FTP(?:Account)?|HTTP|I(?:MAP|RC)|LDAP|NNTP|POP3|S(?:MTP|OCKS)|Telnet))|ER(?:N(?:C(?:rossStream(?:ResetNote)?|urrentVersion)|FormatMask|IndexArray|Line(?:EndKerning|Start)|No(?:CrossKerning|StakeNote|t(?:Applied|esRequested))|OrderedList|ResetCrossStream|S(?:impleArray|tateTable)|Tag|UnusedBits|V(?:ariation|ertical))|X(?:Action(?:OffsetMask|Type(?:AnchorPoints|Co(?:ntrolPoints|ordinates)|Mask))|C(?:ontrolPoint|rossStream(?:ResetNote)?|urrentVersion)|Descending|FormatMask|IndexArray|Line(?:EndKerning|Start)|No(?:CrossKerning|StakeNote|t(?:Applied|esRequested))|OrderedList|ResetCrossStream|S(?:impleArray|tateTable)|Tag|Unused(?:Bits|Flags)|V(?:a(?:luesAreLong|riation)|ertical)))|L(?:GroupIdentifier|I(?:con|dentifier)|K(?:CHR(?:Data|Kind|uchrKind)|ind)|L(?:anguageCode|ocalizedName)|Name|USKeyboard|uchr(?:Data|Kind))|a(?:na(?:SpacingType|ToRomanizationSelector)|takanaToHiraganaSelector)|e(?:epArrangedIcon|rnelExtensionsFolderType|y(?:board(?:ANSI|I(?:SO|nputMethodClass)|JIS|Layout(?:Icon|sFolderType)|Unknown)|chain(?:FolderType|ListChangedKCEvent))))|L(?:A(?:AllMorphemes|DefaultEdge|EndOfSourceTextMask|FreeEdge|IncompleteEdge|MorphemesArrayVersion|Speech(?:BagyouGodan|Chimei(?:Setsubigo)?|Do(?:kuritsugo|ushi)|Fu(?:kushi|tsuuMeishi)|G(?:agyouGodan|odanDoushi)|IchidanDoushi|J(?:inmei(?:Mei|Se(?:i|tsubigo))?|o(?:doushi|shi))|K(?:a(?:gyouGodan|henDoushi|ndoushi|tsuyou(?:Gokan|Katei|M(?:ask|eirei|izen)|Ren(?:tai|you)|Syuushi))|ei(?:douMeishi|you(?:doushi|shi))|igou|oyuuMeishi|uten)|M(?:agyouGodan|e(?:diumClassMask|ishi)|uhinshi)|NagyouGodan|R(?:agyouGodan|entaishi|oughClassMask)|S(?:a(?:gyouGodan|hen(?:Doushi|Meishi))|e(?:iku|t(?:su(?:bi(?:Chimei|go)|zokushi)|tougo))|oshikimei(?:Setsubigo)?|trictClassMask|uu(?:jiSet(?:subigo|tougo)|shi))|T(?:a(?:gyouGodan|nkanji)|outen)|WagyouGodan|ZahenDoushi))|CAR(?:C(?:tlPointFormat|urrentVersion)|LinearFormat|Tag)|S(?:A(?:ccept(?:AllowLoginUI|Default)|pp(?:DoesNot(?:ClaimTypeErr|SupportSchemeWarning)|InTrashErr|licationNotFoundErr)|ttributeNot(?:FoundErr|SettableErr))|CannotSetInfoErr|Data(?:Err|TooOldErr|UnavailableErr)|ExecutableIncorrectFormat|GarbageCollectionUnsupportedErr|Incompatible(?:ApplicationVersionErr|SystemVersionErr)|Launch(?:A(?:nd(?:DisplayErrors|Hide(?:Others)?|Print)|sync)|D(?:efaults|ont(?:AddToRecents|Switch))|InProgressErr|NewInstance)|MultipleSessionsNotSupportedErr|No(?:32BitEnvironmentErr|ClassicEnvironmentErr|ExecutableErr|LaunchPermissionErr|R(?:egistrationInfoErr|osettaEnvironmentErr)|t(?:AnApplicationErr|InitializedErr|RegisteredErr))|Roles(?:All|Editor|None|Shell|Viewer)|S(?:erverCommunicationErr|haredFileList(?:DoNotMountVolumes|NoUserInteraction))|Unknown(?:Creator|Err|Type(?:Err)?))|TAGCurrentVersion|a(?:belKCItemAttr|nguageTagType|rge(?:1BitMask|32BitData|4Bit(?:Data|IconSize)|8Bit(?:Data|IconSize|Mask)|IconSize)|st(?:DomainConstant|FeatureType|IOKitNotificationType|MagicBusyFiletype)|unch(?:ToGetTerminology|erItemsFolderType))|e(?:ft(?:ArrowCharCode|ToRight)|tterCaseType)|i(?:braryAssistantsFolderType|gaturesType|miterParam_(?:AttackTime|DecayTime|PreGain)|n(?:e(?:F(?:eedCharCode|inalSwashesO(?:ffSelector|nSelector))|InitialSwashesO(?:ffSelector|nSelector)|arPCMFormatFlag(?:Is(?:AlignedHigh|BigEndian|Float|Non(?:Interleaved|Mixable)|Packed|SignedInteger)|s(?:AreAllClear|SampleFraction(?:Mask|Shift))))|guisticRearrangement(?:O(?:ffSelector|nSelector)|Type))|stDef(?:ProcPtr|Standard(?:IconType|TextType)|UserProcType))|o(?:c(?:al(?:Domain|PPDDomain|e(?:A(?:llPartsMask|ndVariantNameMask)|Language(?:Mask|VariantMask)|NameMask|OperationVariantNameMask|Region(?:Mask|VariantMask)|Script(?:Mask|VariantMask)|s(?:BufferTooSmallErr|DefaultDisplayStatus|Folder(?:Icon|Type)|TableFormatErr)))|k(?:KCEvent(?:Mask)?|ed(?:BadgeIcon|Icon)))|g(?:osO(?:ffSelector|nSelector)|sFolderType)|w(?:PassParam_(?:CutoffFrequency|Resonance)|erCase(?:NumbersSelector|PetiteCapsSelector|SmallCapsSelector|Type))))|M(?:68kISA|D(?:Label(?:LocalDomain|UserDomain)|Query(?:AllowFSTranslation|ReverseSortOrderFlag|Synchronous|WantsUpdates))|IDI(?:DriversFolderType|I(?:DNotUnique|nvalid(?:Client|Port|UniqueID))|M(?:essageSendErr|sg(?:IOError|Object(?:Added|Removed)|PropertyChanged|Se(?:rialPortOwnerChanged|tupChanged)|ThruConnectionsChanged))|No(?:C(?:onnection|urrentSetup)|tPermitted)|Object(?:NotFound|Type_(?:De(?:stination|vice)|E(?:ntity|xternal(?:De(?:stination|vice)|Entity|Source))|Other|Source))|Se(?:rverStartErr|tupFormatErr)|Unknown(?:E(?:ndpoint|rror)|Property)|Wrong(?:EndpointType|PropertyType|Thread))|OR(?:T(?:C(?:o(?:ntextualType|ver(?:Descending|IgnoreVertical|TypeMask|Vertical))|urr(?:Insert(?:Before|Count(?:Mask|Shift)|KashidaLike)|JustTableCount(?:Mask|Shift)|entVersion))|DoInsertionsBefore|I(?:nsertion(?:Type|sCountMask)|sSplitVowelPiece)|Lig(?:FormOffset(?:Mask|Shift)|LastAction|StoreLigature|atureType)|Mark(?:Insert(?:Before|Count(?:Mask|Shift)|KashidaLike)|JustTableCount(?:Mask|Shift))|RearrangementType|SwashType|Tag|ra(?:CDx(?:A(?:B)?|BA)?|D(?:Cx(?:A(?:B)?|BA)?|x(?:A(?:B)?|BA)?)|NoAction|x(?:A(?:B)?|BA)))|X(?:C(?:over(?:Descending|IgnoreVertical|LogicalOrder|TypeMask|Vertical)|urrentVersion)|Tag))|P(?:A(?:ddressSpaceInfoVersion|llocate(?:1(?:024ByteAligned|6ByteAligned)|32ByteAligned|4096ByteAligned|8ByteAligned|AltiVecAligned|ClearMask|DefaultAligned|GloballyMask|InterlockAligned|MaxAlignment|No(?:CreateMask|GrowthMask)|ResidentMask|VM(?:PageAligned|XAligned))|nyRemoteContext|syncInterruptRemoteContext)|BlueBlockingErr|Cr(?:eateTask(?:NotDebuggableMask|SuspendedMask|TakesAllExceptionsMask|ValidOptionsMask)|iticalRegionInfoVersion)|DeletedErr|E(?:G4Object_(?:AAC_(?:L(?:C|TP)|Main|S(?:BR|SR|calable))|CELP|HVXC|TwinVQ)|ventInfoVersion)|HighLevelDebugger|I(?:n(?:sufficientResourcesErr|terruptRemoteContext|validIDErr)|terationEndErr)|LowLevelDebugger|M(?:axAllocSize|idLevelDebugger)|N(?:anokernelNeedsMemoryErr|o(?:ID|tificationInfoVersion))|OwningProcessRemoteContext|Pr(?:eserveTimerIDMask|ivilegedErr|ocess(?:CreatedErr|TerminatedErr))|QueueInfoVersion|SemaphoreInfoVersion|T(?:ask(?:AbortedErr|Blocked(?:Err)?|CreatedErr|InfoVersion|Propagate(?:Mask)?|R(?:e(?:ady|sume(?:Branch(?:Mask)?|Mask|Step(?:Mask)?))|unning)|St(?:ate(?:32BitMemoryException|FPU|Machine|Registers|TaskInfo|Vectors)|oppedErr))|ime(?:IsD(?:eltaMask|urationMask)|outErr)))|a(?:c(?:Arabic(?:AlBayanVariant|StandardVariant|T(?:huluthVariant|rueTypeVariant))|C(?:roatian(?:CurrencySignVariant|DefaultVariant|EuroSignVariant)|yrillic(?:CurrSign(?:StdVariant|UkrVariant)|DefaultVariant|EuroSignVariant))|Farsi(?:StandardVariant|TrueTypeVariant)|Greek(?:DefaultVariant|EuroSignVariant|NoEuroSignVariant)|He(?:brew(?:FigureSpaceVariant|StandardVariant)|lpVersion)|Icelandic(?:St(?:andardVariant|d(?:CurrSignVariant|DefaultVariant|EuroSignVariant))|T(?:T(?:CurrSignVariant|DefaultVariant|EuroSignVariant)|rueTypeVariant))|Japanese(?:BasicVariant|PostScript(?:PrintVariant|ScrnVariant)|St(?:andardVariant|dNoVerticalsVariant)|VertAtKuPlusTenVariant)|MemoryMaximumMemoryManagerBlockSize|OSReadMe(?:FolderIcon|sFolderType)|Roman(?:CurrencySignVariant|DefaultVariant|EuroSignVariant|Latin1(?:CroatianVariant|DefaultVariant|IcelandicVariant|RomanianVariant|StandardVariant|TurkishVariant)|StandardVariant|ian(?:CurrencySignVariant|DefaultVariant|EuroSignVariant))|VT100(?:CurrencySignVariant|DefaultVariant|EuroSignVariant)|hineNameStrID)|gic(?:BusyCreationDate|TemporaryItemsFolderType)|le|nagedItemsFolderType|t(?:h(?:SymbolsSelector|ematical(?:ExtrasType|GreekO(?:ffSelector|nSelector)))|rixMixerParam_(?:Enable|P(?:ost(?:AveragePower(?:Linear)?|PeakHoldLevel(?:Linear)?)|re(?:AveragePower(?:Linear)?|PeakHoldLevel(?:Linear)?))|Volume))|x(?:AsyncArgs|InputLengthOfAppleJapaneseEngine|K(?:anjiLengthInAppleJapaneseDictionary|eyLength)|YomiLengthInAppleJapaneseDictionary|imumBlocksIn4GB))|enu(?:A(?:ppleLogo(?:FilledGlyph|OutlineGlyph)|ttr(?:AutoDisable|CondenseSeparators|DoNot(?:CacheImage|UseUserCommandKeys)|ExcludesMarkColumn|Hidden|UsePencilGlyph))|BlankGlyph|C(?:GImageRefType|a(?:lcItemMsg|psLockGlyph)|heckmarkGlyph|learGlyph|o(?:lorIconType|mmandGlyph|nt(?:ext(?:Co(?:mmandIDSearch|ntextualMenu)|DontUpdate(?:Enabled|Icon|Key|Text)|Inspection|KeyMatching|Menu(?:Bar(?:Tracking)?|Enabling)|P(?:opUp(?:Tracking)?|ullDown)|Submenu|ualMenuGlyph)|rol(?:Glyph|ISOGlyph|Modifier))))|D(?:e(?:f(?:ClassID|ProcPtr)|lete(?:LeftGlyph|RightGlyph))|i(?:amondGlyph|sposeMsg)|own(?:ArrowGlyph|wardArrowDashedGlyph)|raw(?:ItemsMsg|Msg))|E(?:isuGlyph|jectGlyph|nterGlyph|scapeGlyph|vent(?:DontCheckSubmenus|IncludeDisabledItems|QueryOnly))|F(?:1(?:0Glyph|1Glyph|2Glyph|3Glyph|4Glyph|5Glyph|6Glyph|7Glyph|8Glyph|9Glyph|Glyph)|2Glyph|3Glyph|4Glyph|5Glyph|6Glyph|7Glyph|8Glyph|9Glyph|indItemMsg)|H(?:elpGlyph|iliteItemMsg)|I(?:con(?:Re(?:fType|sourceType)|SuiteType|Type)|nitMsg|tem(?:Attr(?:Auto(?:Disable|Repeat)|CustomDraw|D(?:isabled|ynamic)|Hidden|I(?:conDisabled|gnoreMeta|ncludeInCmdKeyMatching)|NotPreviousAlternate|S(?:e(?:ctionHeader|parator)|ubmenuParentChoosable)|U(?:pdateSingleItem|seVirtualKey))|Data(?:A(?:llDataVersion(?:One|T(?:hree|wo))|ttribute(?:dText|s))|C(?:FString|md(?:Key(?:Glyph|Modifiers)?|VirtualKey)|ommandID)|Enabled|Font(?:ID)?|I(?:con(?:Enabled|Handle|ID)|ndent)|Mark|Properties|Refcon|S(?:tyle|ubmenu(?:Handle|ID))|Text(?:Encoding)?)))|KanaGlyph|Left(?:Arrow(?:DashedGlyph|Glyph)|DoubleQuotesJapaneseGlyph)|N(?:o(?:CommandModifier|Icon|Modifiers|nmarkingReturnGlyph|rthwestArrowGlyph)|ullGlyph)|Option(?:Glyph|Modifier)|P(?:a(?:ge(?:DownGlyph|UpGlyph)|ragraphKoreanGlyph)|encilGlyph|o(?:pUpMsg|werGlyph)|ropertyPersistent)|R(?:eturn(?:Glyph|R2LGlyph)|ight(?:Arrow(?:DashedGlyph|Glyph)|DoubleQuotesJapaneseGlyph))|S(?:h(?:ift(?:Glyph|Modifier)|rinkIconType)|izeMsg|mallIconType|outheastArrowGlyph|paceGlyph|tdMenu(?:BarProc|Proc)|ystemIconSelectorType)|T(?:ab(?:LeftGlyph|RightGlyph)|hemeSavvyMsg|ra(?:ckingMode(?:Keyboard|Mouse)|demarkJapaneseGlyph))|UpArrow(?:DashedGlyph|Glyph))|i(?:crosecondScale|llisecondScale|ni(?:1BitMask|4BitData|8BitData))|o(?:d(?:DateKCItemAttr|al(?:DialogVariantCode|WindowClass)|em(?:OutOfMemory|PreferencesMissing|Script(?:Missing|sFolderType)))|nospaced(?:NumbersSelector|TextSelector)|u(?:nted(?:BadgeIcon|Folder(?:AliasType|Icon(?:Resource)?))|se(?:Params(?:ClickAndHold|DragInitiation|ProxyIcon|Sticky)|Tracking(?:ClientEvent|KeyModifiersChanged|Mouse(?:D(?:own|ragged)|E(?:ntered|xited)|Moved|Pressed|Released|Up)|ScrollWheel|TimedOut|UserCancelled)|UpOutOfSlop))|v(?:able(?:Alert(?:VariantCode|WindowClass)|Modal(?:DialogVariantCode|WindowClass))|ieDocumentsFolderType))|u(?:lti(?:ChannelMixerParam_(?:Enable|P(?:an|ost(?:AveragePower|PeakHoldLevel)|re(?:AveragePower|PeakHoldLevel))|Volume)|band(?:CompressorParam_(?:AttackTime|C(?:ompressionAmount(?:1|2|3|4)|rossover(?:1|2|3))|EQ(?:1|2|3|4)|Headroom(?:1|2|3|4)|InputAmplitude(?:1|2|3|4)|OutputAmplitude(?:1|2|3|4)|P(?:ostgain|regain)|ReleaseTime|Threshold(?:1|2|3|4))|Filter_(?:Bandwidth(?:1|2|3)|Center(?:Freq(?:1|2|3)|Gain(?:1|2|3))|High(?:F(?:ilterType|requency)|Gain)|Low(?:F(?:ilterType|requency)|Gain)))|processingFolderType)|sic(?:D(?:evice(?:MIDIEventSelect|P(?:aram_(?:ReverbVolume|Tuning|Volume)|r(?:epareInstrumentSelect|operty_(?:BankName|DualSchedulingMode|GroupOutputBus|Instrument(?:Count|N(?:ame|umber))|MIDIXMLNames|PartGroup|S(?:oundBank(?:Data|FS(?:Ref|Spec)|URL)|treamFromDisk|upportsStartStopNote)|UsesInternalReverb)))|R(?:ange|eleaseInstrumentSelect)|S(?:ampleFrameMask_(?:IsScheduled|SampleOffset)|t(?:artNoteSelect|opNoteSelect)|ysExSelect))|ocumentsFolderType)|EventType_(?:AUPreset|Extended(?:Control|Note|Tempo)|M(?:IDI(?:ChannelMessage|NoteMessage|RawData)|eta)|NULL|Parameter|User)|NoteEvent_U(?:nused|seGroupInstrument)|Sequence(?:File(?:Flags_(?:Default|EraseFile)|_(?:AnyType|MIDIType|iMelodyType))|LoadSMF_(?:ChannelsToTracks|PreserveTracks)|Type_(?:Beats|S(?:amples|econds))))))|N(?:LCCharactersSelector|S(?:L(?:68kContextNotSupported|B(?:ad(?:ClientInfoPtr|DataTypeErr|NetConnection|ProtocolTypeErr|ReferenceErr|ServiceTypeErr|URLSyntax)|ufferTooSmallForData)|CannotContinueLookup|ErrNullPtrError|In(?:itializationFailed|sufficient(?:OTVer|SysVer)|validPluginSpec)|N(?:o(?:C(?:arbonLib|ontextAvailable)|ElementsInList|PluginsFo(?:rSearch|und)|SupportForService|tI(?:mplementedYet|nitialized))|ull(?:ListPtr|NeighborhoodPtr))|PluginLoadFailed|RequestBufferAlreadyInList|S(?:chedulerError|earchAlreadyInProgress|omePluginsFailedToLoad)|UILibraryNotAvailable)|p(?:A(?:dd(?:PlayerFailedErr|ressInUseErr)|lready(?:AdvertisingErr|InitializedErr))|C(?:antBlockErr|onnectFailedErr|reateGroupFailedErr)|F(?:eatureNotImplementedErr|reeQExhaustedErr)|GameTerminatedErr|HostFailedErr|In(?:itializationFailedErr|valid(?:AddressErr|DefinitionErr|G(?:ameRefErr|roupIDErr)|P(?:arameterErr|layerIDErr|rotocol(?:ListErr|RefErr))))|JoinFailedErr|Me(?:mAllocationErr|ssageTooBigErr)|N(?:ameRequiredErr|o(?:GroupsErr|HostVolunteersErr|PlayersErr|tAdvertisingErr))|OT(?:NotPresentErr|VersionTooOldErr)|P(?:ipeFullErr|rotocolNotAvailableErr)|RemovePlayerFailedErr|SendFailedErr|T(?:imeoutErr|opologyNotSupportedErr)))|a(?:meLocked|nosecondScale|v(?:CustomControlMessageFailedErr|Invalid(?:CustomControlMessageErr|SystemConfigErr)|MissingKindStringErr|WrongDialog(?:ClassErr|StateErr)))|e(?:gativeKCItemAttr|twork(?:Domain|PPDDomain)|ut(?:er|ralScript)|verAuthenticate|w(?:DebugHeap|S(?:izeParameter|tyleHeap|uspend)|TimePitchParam_(?:EnablePeakLocking|Overlap|Pitch|Rate))|xt(?:Body|WindowGroup))|o(?:A(?:lternatesSelector|nnotationSelector)|ByteCode|C(?:JK(?:ItalicRomanSelector|SymbolAlternativesSelector)|ard(?:BusCISErr|E(?:nablersFoundErr|rr)|SevicesSocketsErr)|lientTableErr|o(?:mpatibleNameErr|nstraint))|En(?:ablerForCardErr|dingProsody)|F(?:ilesIcon|olderIcon|ractionsSelector)|I(?:OWindowRequestedErr|deographicAlternativesSelector)|More(?:I(?:nterruptSlotsErr|temsErr)|TimerClientsErr)|OrnamentsSelector|Process|RubyKanaSelector|S(?:peechInterrupt|tyl(?:eOptionsSelector|isticAlternatesSelector)|uchPowerSource)|T(?:hreadID|imeOut|rans(?:form|literationSelector))|UserAuthentication|WriteIcon|n(?:BreakingSpaceCharCode|FinalSwashesO(?:ffSelector|nSelector)|eKCStopOn)|rmalPositionSelector|t(?:Paged|ReadyErr|ZVCapableErr|eIcon))|u(?:llCharCode|m(?:AUNBandEQFilterTypes|ber(?:C(?:aseType|tlCTabEntries)|OfResponseFrequencies|SpacingType))))|O(?:CRInputMethodClass|PBD(?:C(?:ontrolPointFormat|urrentVersion)|DistanceFormat|Tag)|S(?:A(?:C(?:anGetSource|omponentType)|DontUsePhac|Error(?:A(?:pp|rgs)|BriefMessage|ExpectedType|Message|Number|OffendingObject|PartialResult|Range)|FileType|GenericScriptingComponentSubtype|Mode(?:A(?:lwaysInteract|ugmentContext)|C(?:an(?:Interact|tSwitchLayer)|ompileIntoContext)|D(?:isp(?:atchToDirectObject|layForHumans)|o(?:Record|nt(?:Define|GetDataForArguments|Reconnect|StoreParent)))|FullyQualifyDescriptors|N(?:everInteract|ull)|PreventGetSource)|N(?:oDispatch|ull(?:Mode|Script))|RecordedText|S(?:cript(?:BestType|Is(?:Modified|Type(?:CompiledScript|Script(?:Context|Value)))|ResourceType)|elect(?:AvailableDialect(?:CodeList|s)|Co(?:erce(?:FromDesc|ToDesc)|mp(?:ile(?:Execute)?|onentSpecificStart)|py(?:DisplayString|ID|S(?:cript|ourceString)))|D(?:isp(?:lay|ose)|o(?:Event|Script))|Execute(?:Event)?|Get(?:ActiveProc|C(?:reateProc|urrentDialect)|DialectInfo|ResumeDispatchProc|S(?:criptInfo|endProc|ource))|Load(?:Execute)?|MakeContext|S(?:cript(?:Error|ingComponentName)|et(?:ActiveProc|C(?:reateProc|urrentDialect)|DefaultTarget|ResumeDispatchProc|S(?:criptInfo|endProc))|t(?:artRecording|o(?:pRecording|re))))|u(?:ite|pports(?:AE(?:Coercion|Sending)|Co(?:mpiling|nvenience)|Dialects|EventHandling|GetSource|Recording)))|UseStandardDispatch|sync(?:CompleteMessageID|Ref(?:64(?:Count|Size)|Count|Size)))|IZ(?:CodeInSharedLibraries|DontOpenResourceFile|OpenWithReadPermission|dontAcceptRemoteEvents)|NotificationMessageID)|T(?:A(?:ccessErr|ddressBusyErr)|B(?:ad(?:AddressErr|ConfigurationErr|DataErr|FlagErr|NameErr|OptionErr|QLenErr|ReferenceErr|S(?:equenceErr|yncErr))|ufferOverflowErr)|C(?:anceledErr|lientNotInittedErr|onfigurationChangedErr)|DuplicateFoundErr|FlowErr|IndOutErr|LookErr|No(?:AddressErr|D(?:ataErr|isconnectErr)|Error|ReleaseErr|StructureTypeErr|UDErrErr|t(?:FoundErr|SupportedErr))|Out(?:OfMemoryErr|StateErr)|P(?:ort(?:HasDiedErr|LostConnection|WasEjectedErr)|ro(?:tocolErr|viderMismatchErr))|QFullErr|Res(?:AddressErr|QLenErr)|S(?:tateChangeErr|ysErrorErr)|UserRequestedErr)|ff(?:linePreflight_(?:NotRequired|Optional|Required)|set2Pos)|ld68kRTA|n(?:AppropriateDisk|SystemDisk|eByteCode)|p(?:aque(?:A(?:ddressSpaceID|nyID|reaID)|C(?:o(?:herenceID|nsoleID)|puID|riticalRegionID)|EventID|NotificationID|ProcessID|QueueID|SemaphoreID|T(?:askID|imerID))|en(?:D(?:oc(?:EditorsFolderType|FolderType|LibrariesFolderType|ShellPlugInsFolderType)|ropIconVariant)|FolderIcon(?:Resource)?|IconVariant)|tionUnicode)|r(?:Connections|dinalsSelector|namentSetsType)|therPluginFormat_(?:AU|Undefined|k(?:MAS|VST))|ut(?:OfResourceErr|putTextInUnicodeEncoding(?:Bit|Mask))|verla(?:ppingCharactersType|yWindowClass)|wne(?:dFolderIcon(?:Resource)?|r(?:I(?:D2Name|con)|Name2ID)))|P(?:CSTo(?:Device|PCS)|EF(?:AbsoluteExport|Co(?:deS(?:ection|ymbol)|nstantSection)|D(?:ataSymbol|ebugSection)|Ex(?:ceptionSection|ecDataSection|pSym(?:ClassShift|MaxNameOffset|NameOffsetMask))|FirstSectionHeaderOffset|Gl(?:obalShare|ueSymbol)|Hash(?:LengthShift|MaxLength|Slot(?:FirstKeyMask|Max(?:KeyIndex|SymbolCount)|SymCountShift)|ValueMask)|I(?:mpSym(?:ClassShift|MaxNameOffset|NameOffsetMask)|nitLibBeforeMask)|LoaderSection|P(?:ackedDataSection|kData(?:Block|Count5Mask|MaxCount5|OpcodeShift|Repeat(?:Block|Zero)?|VCount(?:EndMask|Mask|Shift)|Zero)|ro(?:cessShare|tectedShare))|Re(?:exportedImport|loc(?:B(?:asicOpcodeRange|ySect(?:C|D(?:WithSkip)?))|I(?:mportRun|ncrPosition(?:MaxOffset)?)|Lg(?:By(?:Import(?:MaxIndex)?|SectionSubopcode)|Repeat(?:Max(?:ChunkCount|RepeatCount))?|Set(?:OrBySection(?:MaxIndex)?|Sect(?:CSubopcode|DSubopcode)))|RunMaxRunLength|S(?:etPos(?:MaxOffset|ition)|m(?:By(?:Import|Section)|IndexMaxIndex|Repeat(?:Max(?:ChunkCount|RepeatCount))?|SetSect(?:C|D)))|TVector(?:12|8)|UndefinedOpcode|VTable8|WithSkipMax(?:RelocCount|SkipCount)))|T(?:OCSymbol|VectorSymbol|ag(?:1|2)|racebackSection)|Un(?:definedSymbol|packedDataSection)|Version|WeakImport(?:LibMask|SymMask))|M(?:AllocationFailure|Border(?:Double(?:Hairline|Thickline)|Single(?:Hairline|Thickline))|C(?:MYKColorSpaceModel|VMSymbolNotFound|ancel|loseFailed|overPage(?:After|Before|None)|reateMessageFailed)|D(?:ataFormatXML(?:Compressed|Default|Minimal)|e(?:leteSubTicketFailed|stination(?:F(?:ax|ile)|Invalid|Pr(?:eview|inter|ocessPDF))|vNColorSpaceModel)|o(?:cumentNotFound|ntSwitchPDEError)|uplex(?:No(?:Tumble|ne)|Tumble))|EditRequestFailed|F(?:eatureNotInstalled|ileOrDirOperationFailed|ontN(?:ameTooLong|otFound))|G(?:eneral(?:CGError|Error)|rayColorSpaceModel)|HideInlineItems|I(?:O(?:AttrNotAvailable|MSymbolNotFound)|n(?:ternalError|valid(?:Allocator|C(?:VMContext|alibrationTarget|onnection)|FileType|I(?:OMContext|ndex|tem)|Job(?:ID|Template)|Key|LookupSpec|Object|P(?:BMRef|DEContext|MContext|a(?:geFormat|per|rameter)|r(?:eset|int(?:Se(?:ssion|ttings)|er(?:Address|Info)?)))|Reply|S(?:tate|ubTicket)|T(?:icket|ype)|Value))|temIsLocked)|Job(?:Busy|Canceled|GetTicket(?:BadFormatError|ReadError)|ManagerAborted|NotFound|Stream(?:EndError|OpenFailed|ReadFailed))|Key(?:Not(?:Found|Unique)|OrValueNotFound)|La(?:ndscape|stErrorCodeToMakeMaintenanceOfThisListEasier|yout(?:BottomTop(?:LeftRight|RightLeft)|LeftRight(?:BottomTop|TopBottom)|RightLeft(?:BottomTop|TopBottom)|TopBottom(?:LeftRight|RightLeft)))|MessagingError|No(?:Default(?:Item|Printer|Settings)|Error|PrinterJobID|S(?:electedPrinters|uchEntry)|tImplemented)|O(?:bjectInUse|penFailed|utOfScope)|P(?:MSymbolNotFound|a(?:geToPaperMapping(?:None|ScaleToFit)|perType(?:Coated|Glossy|P(?:lain|remium)|T(?:Shirt|ransparency)|Unknown))|ermissionError|lugin(?:NotFound|RegisterationFailed)|ortrait|r(?:BrowserNoUI|int(?:AllPages|er(?:Idle|Processing|Stopped))))|Qu(?:ality(?:Best|Draft|Highest|InkSaver|Lowest|Normal|Photo)|eue(?:AlreadyExists|JobFailed|NotFound))|R(?:GBColorSpaceModel|e(?:ad(?:Failed|GotZeroData)|verse(?:Landscape|Portrait)))|S(?:caling(?:CenterOn(?:ImgArea|Paper)|Pin(?:Bottom(?:Left|Right)|Top(?:Left|Right)))|erver(?:A(?:lreadyRunning|ttributeRestricted)|CommunicationFailed|NotFound|Suspended)|how(?:DefaultInlineItems|Inline(?:Copies|Orientation|Pa(?:geRange(?:WithSelection)?|perSize)|Scale)|PageAttributesPDE)|implexTumble|t(?:atusFailed|ringConversionFailure)|ubTicketNotFound|yncRequestFailed)|T(?:emplateIsLocked|icket(?:IsLocked|TypeNotFound))|U(?:n(?:ableToFindProcess|expectedImagingError|known(?:ColorSpaceModel|DataType|Message)|locked|supportedConnection)|pdateTicketFailed|serOrGroupNotFound)|Val(?:idateTicketFailed|ueOutOfRange)|WriteFailed|XMLParseError)|OSIXError(?:Base|E(?:2BIG|A(?:CCES|DDR(?:INUSE|NOTAVAIL)|FNOSUPPORT|GAIN|LREADY|UTH)|B(?:AD(?:ARCH|EXEC|F|M(?:ACHO|SG)|RPC)|USY)|C(?:ANCELED|HILD|ONN(?:ABORTED|RE(?:FUSED|SET)))|D(?:E(?:ADLK|STADDRREQ|VERR)|OM|QUOT)|EXIST|F(?:AULT|BIG|TYPE)|HOST(?:DOWN|UNREACH)|I(?:DRM|LSEQ|N(?:PROGRESS|TR|VAL)|O|S(?:CONN|DIR))|LOOP|M(?:FILE|LINK|SGSIZE|ULTIHOP)|N(?:AMETOOLONG|E(?:EDAUTH|T(?:DOWN|RESET|UNREACH))|FILE|O(?:ATTR|BUFS|D(?:ATA|EV)|E(?:NT|XEC)|L(?:CK|INK)|M(?:EM|SG)|PROTOOPT|S(?:PC|R|TR|YS)|T(?:BLK|CONN|DIR|EMPTY|S(?:OCK|UP)|TY))|XIO)|O(?:PNOTSUPP|VERFLOW)|P(?:ERM|FNOSUPPORT|IPE|RO(?:C(?:LIM|UNAVAIL)|G(?:MISMATCH|UNAVAIL)|TO(?:NOSUPPORT|TYPE)?)|WROFF)|R(?:ANGE|EMOTE|OFS|PCMISMATCH)|S(?:H(?:LIBVERS|UTDOWN)|OCKTNOSUPPORT|PIPE|RCH|TALE)|T(?:IME(?:DOUT)?|OOMANYREFS|XTBSY)|USERS|XDEV))|ROP(?:A(?:LDirectionClass|NDirectionClass)|BNDirectionClass|C(?:SDirectionClass|anHang(?:LTMask|RBMask)|urrentVersion)|DirectionMask|E(?:NDirectionClass|SDirectionClass|TDirectionClass)|IsFloaterMask|L(?:DirectionClass|R(?:EDirectionClass|ODirectionClass))|N(?:SMDirectionClass|umDirectionClasses)|ONDirectionClass|P(?:DFDirectionClass|SDirectionClass|airOffset(?:Mask|S(?:hift|ign)))|R(?:DirectionClass|L(?:EDirectionClass|ODirectionClass)|ightConnectMask)|S(?:DirectionClass|ENDirectionClass)|Tag|UseRLPairMask|WSDirectionClass|ZeroReserved)|a(?:ckageAliasType|ge(?:DownCharCode|InMemory|OnDisk|UpCharCode)|nn(?:erParam_(?:Azimuth|CoordScale|Distance|Elevation|Gain|RefDistance)|ingMode_(?:SoundField|VectorBasedPanning))|r(?:amet(?:erEvent_(?:Immediate|Ramped)|ricEQParam_(?:CenterFreq|Gain|Q))|enthesisAnnotationSelector|tiallyConnectedSelector)|s(?:calStackBased|s(?:CallToChainErr|Selector|word(?:ChangedKCEvent(?:Mask)?)?)|teboard(?:ClientIsOwner|Flavor(?:No(?:Flags|tSaved)|Promised|RequestOnly|S(?:ender(?:Only|Translated)|ystemTranslated))|Modified|StandardLocation(?:Trash|Unknown)))|thKCItemAttr)|e(?:ncil(?:LeftUnicode|Unicode)|riod(?:AnnotationSelector|sToEllipsisO(?:ffSelector|nSelector)))|i(?:CharactersSelector|ctureD(?:ialogItem|ocumentsFolderType))|l(?:ain(?:DialogVariantCode|WindowClass)|otIconRefNo(?:Image|Mask|rmalFlags))|o(?:licyKCStopOn|rtKCItemAttr|s(?:2Offset|tCardEventErr)|wer(?:Handler(?:ExistsForDeviceErr|NotFoundFor(?:DeviceErr|ProcErr))|Mgt(?:MessageNotHandled|RequestDenied)|PC(?:ISA|RTA)))|r(?:e(?:MacOS91(?:A(?:ppl(?:eExtrasFolderType|icationsFolderType)|ssistantsFolderType|utomountedServersFolderType)|In(?:stallerLogsFolderType|ternetFolderType)|MacOSReadMesFolderType|StationeryFolderType|UtilitiesFolderType)|emptiveThread|f(?:erence(?:PanesFolderType|sFolder(?:AliasType|Icon(?:Resource)?|Type))|lightThenPause)|v(?:entOverlapO(?:ffSelector|nSelector)|ious(?:Body|WindowGroup)))|i(?:nt(?:Monitor(?:DocsFolder(?:AliasType|Type)|FolderIcon(?:Resource)?)|er(?:D(?:escriptionFolder(?:Icon|Type)|riverFolder(?:Icon|Type))|sFolderType)|ingPlugInsFolderType)|v(?:ateF(?:olderIcon(?:Resource)?|rameworksFolderType)|ilegeViolationException))|o(?:c(?:DescriptorIs(?:Absolute|Index|ProcPtr|Relative)|ess(?:DictionaryIncludeAllInformationMask|TransformTo(?:BackgroundApplication|ForegroundApplication|UIElementApplication)|orTempRoutineRequiresMPLib2))|gramTargetLevel_(?:Minus(?:2(?:0dB|3dB)|31dB)|None)|portional(?:CJKRomanSelector|IdeographsSelector|KanaSelector|NumbersSelector|TextSelector)|t(?:ected(?:ApplicationFolderIcon|SystemFolderIcon)|ocolKCItemAttr)))|ublic(?:Folder(?:Icon|Type)|KeyHashKCItemAttr|ThemeFontCount))|Q(?:D(?:C(?:orruptPICTDataErr|ursor(?:AlreadyRegistered|NotRegistered))|No(?:ColorHWCursorSupport|Palette))|LPreviewPDF(?:PagesWithThumbnailsOn(?:LeftStyle|RightStyle)|StandardStyle)|TSSUnknownErr|u(?:arterWidth(?:NumbersSelector|TextSelector)|estionMarkIcon|i(?:ck(?:LookFolderType|Time(?:ComponentsFolderType|ExtensionsFolderType))|t(?:AtNormalTimeMask|Before(?:FBAsQuitMask|NormalTimeMask|ShellQuitsMask|TerminatorAppQuitsMask)|N(?:everMask|otQuitDuring(?:InstallMask|LogoutMask))|OptionsMask))))|R(?:A(?:ATalkInactive|C(?:allBackFailed|on(?:figurationDBInitErr|nectionCanceled))|DuplicateIPAddr|ExtAuthenticationFailed|In(?:compatiblePrefs|itOpenTransportFailed|stallationDamaged|ternalError|valid(?:P(?:a(?:rameter|ssword)|ort(?:State)?)|SerialProtocol))|MissingResources|N(?:CPRejectedbyPeer|ot(?:Connected|Enabled|PrimaryInterface|Supported))|OutOfMemory|P(?:PP(?:AuthenticationFailed|NegotiationFailed|P(?:eerDisconnected|rotocolRejected)|UserDisconnected)|eerNotResponding|ort(?:Busy|SetupFailed))|RemoteAccessNotReady|StartupFailed|TCPIP(?:Inactive|NotConfigured)|U(?:nknown(?:PortState|User)|ser(?:InteractionRequired|LoginDisabled|Pwd(?:ChangeRequired|EntryRequired))))|a(?:dioButtonDialogItem|ndomParam_(?:Bound(?:A|B)|Curve)|reLigaturesO(?:ffSelector|nSelector))|dPermKCStatus|e(?:ad(?:ExtensionTermsMask|FailureErr|OnlyMemoryException|Reference|yThreadState)|busPicturesO(?:ffSelector|nSelector)|cent(?:ApplicationsFolder(?:Icon|Type)|DocumentsFolder(?:Icon|Type)|ItemsIcon|ServersFolder(?:Icon|Type))|d(?:irectedRelativeFolder|rawHighlighting)|gister(?:A(?:0|1|2|3|4|5|6)|Based|D(?:0|1|2|3|4|5|6|7)|Parameter(?:Mask|Phase|Size(?:Phase|Width)|W(?:hich(?:Phase|Width)|idth))|ResultLocation(?:Phase|Width))|lativeFolder|nderQuality_(?:High|Low|M(?:ax|edium|in))|quiredLigaturesO(?:ffSelector|nSelector)|s(?:FileNotOpened|o(?:lveAlias(?:FileNoUI|TryFileIDFirst)|urceControlDialogItem)|ultSize(?:Mask|Phase|Width))|turn(?:CharCode|Next(?:Group|U(?:G|ser)))|verb(?:2Param_(?:D(?:ecayTimeAt(?:0Hz|Nyquist)|ryWetMix)|Gain|M(?:axDelayTime|inDelayTime)|RandomizeReflections)|Param_(?:DryWetMix|Filter(?:Bandwidth|Enable|Frequency|Gain|Type)|Large(?:Brightness|De(?:lay(?:Range)?|nsity)|Size)|Modulation(?:Depth|Rate)|PreDelay|Small(?:Brightness|De(?:layRange|nsity)|LargeMix|Size))|RoomType_(?:Cathedral|Large(?:Chamber|Hall(?:2)?|Room(?:2)?)|Medium(?:Chamber|Hall(?:2|3)?|Room)|Plate|SmallRoom)))|ight(?:ArrowCharCode|ContainerArrowIcon|ToLeft)|o(?:gerBeepParam_(?:InGateThreshold(?:Time)?|OutGateThreshold(?:Time)?|Roger(?:Gain|Type)|Sensitivity)|lloverIconVariant|man(?:NumeralAnnotationSelector|izationTo(?:HiraganaSelector|KatakanaSelector))|otFolder|u(?:nd(?:TripAACParam_(?:BitRate|CompressedFormatSampleRate|EncodingStrategy|Format|Quality|RateOrQuality)|WindowDefinition|edBoxAnnotationSelector)|tin(?:e(?:DescriptorVersion|Is(?:DispatchedDefaultRoutine|NotDispatchedDefaultRoutine))|gResource(?:ID|Type))))|srcChain(?:AboveA(?:llMaps|pplicationMap)|Below(?:ApplicationMap|SystemMap))|u(?:byKana(?:O(?:ffSelector|nSelector)|Selector|Type)|nningThreadState))|S(?:C(?:BondStatus(?:LinkInvalid|No(?:Partner|tInActiveGroup)|OK|Unknown)|Network(?:Connection(?:Connect(?:ed|ing)|Disconnect(?:ed|ing)|Invalid|PPP(?:Authenticating|Connect(?:ed|ingLink)|Di(?:alOnTraffic|sconnect(?:ed|ingLink))|HoldingLinkOff|Initializing|Negotiating(?:Link|Network)|Suspended|Terminating|WaitingFor(?:CallBack|Redial)))|Flags(?:Connection(?:Automatic|Required)|I(?:nterventionRequired|s(?:Direct|LocalAddress))|Reachable|TransientConnection)|ReachabilityFlags(?:Connection(?:Automatic|On(?:Demand|Traffic)|Required)|I(?:nterventionRequired|s(?:Direct|LocalAddress|WWAN))|Reachable|TransientConnection))|PreferencesNotification(?:Apply|Commit)|Status(?:AccessError|Connection(?:Ignore|NoService)|Failed|InvalidArgument|KeyExists|Locked|MaxLink|N(?:eedLock|o(?:ConfigFile|Key|Link|PrefsSession|StoreSe(?:rver|ssion)|tifierActive))|OK|PrefsBusy|ReachabilityUnknown|Stale))|FNTLookup(?:S(?:egment(?:Array|Single)|i(?:mpleArray|ngleTable))|TrimmedArray|Vector)|K(?:DocumentState(?:AddPending|DeletePending|Indexed|NotIndexed)|Index(?:Inverted(?:Vector)?|Unknown|Vector)|Search(?:BooleanRanked|Option(?:Default|FindSimilar|NoRelevanceScores|SpaceMeansOR)|PrefixRanked|R(?:anked|equiredRanked)))|MPTETime(?:Running|Type(?:2(?:398|4|5|997(?:Drop)?)|30(?:Drop)?|5(?:0|994(?:Drop)?)|60(?:Drop)?)|Unknown|Valid)|O(?:AP(?:1999Schema|2001Schema)|CKS5NoAcceptableMethod)|R(?:A(?:lready(?:Finished|Listening|Released)|utoFinishingParam)|B(?:ad(?:Parameter|Selector)|lock(?:Background|Modally)|ufferTooSmall)|C(?:a(?:llBackParam|n(?:celOnSoundOut|ned22kHzSpeechSource|t(?:Add|GetProperty|ReadLanguageObject|Set(?:DuringRecognition|Property))))|leanupOnClientExit|omponentNotFound)|Default(?:Re(?:cognitionSystemID|jectionLevel)|SpeechSource)|E(?:nabled|xpansionTooDeep)|F(?:eedback(?:AndListeningModes|NotAvail)|oregroundOnly)|Has(?:FeedbackHasListenModes|NoSubItems)|I(?:dleRecognizer|nternalError)|Key(?:Expected|Word)|L(?:MObjType|anguageModel(?:Format|T(?:ooBig|ype))|i(?:stenKey(?:Combo|Mode|Name)|veDesktopSpeechSource))|M(?:odelMismatch|ustCancelSearch)|No(?:ClientLanguageModel|Feedback(?:HasListenModes|NoListenModes)|PendingUtterances|t(?:A(?:RecSystem|SpeechObject|vailable)|FinishedWithRejection|ImplementedYet|ListeningState|if(?:icationParam|yRecognition(?:Beginning|Done))))|O(?:ptional|therRecAlreadyModal|utOfMemory)|P(?:a(?:ramOutOfRange|th(?:Format|Type))|endingSearch|hrase(?:Format|Type))|Re(?:adAudio(?:FSSpec|URL)|cognition(?:Canceled|Done)|fCon|ject(?:able|edWord|ionLevel)|peatable)|S(?:earch(?:InProgress|StatusParam|WaitForAllClients)|ndInSourceDisconnected|oundInVolume|pe(?:edVsAccuracyParam|lling)|ubItemNotFound)|T(?:EXTFormat|ooManyElements)|Use(?:PushToTalk|ToggleListen)|W(?:ants(?:AutoFBGestures|ResultTextDrawn)|ord(?:NotFound|Type)))|S(?:LCiphersuiteGroup(?:ATS(?:Compatibility)?|Compatibility|Default|Legacy)|p(?:CantInstallErr|InternalErr|ParallelUpVectorErr|ScaleToZeroErr|VersionErr))|T(?:Class(?:DeletedGlyph|EndOf(?:Line|Text)|OutOfBounds)|KCrossStreamReset|LigActionMask|MarkEnd|NoAdvance|RearrVerbMask|SetMark|XHasLigAction)|c(?:heduledAudioSliceFlag_(?:BeganToRender(?:Late)?|Complete|Interrupt(?:AtLoop)?|Loop)|ientificInferiorsSelector|r(?:ap(?:ClearNamedScrap|Flavor(?:Mask(?:None|SenderOnly|Translated)|SizeUnknown|Type(?:Movie|Picture|Sound|Text(?:Style)?|U(?:TF16External|nicode(?:Style)?)))|GetNamedScrap|ReservedFlavorType)|eenSaversFolderType|ipt(?:CodeKCItemAttr|ingAdditionsFolder(?:Icon|Type)|sFolder(?:Icon|Type))|oll(?:Bars(?:AlwaysActive|SyncWithFocus)|Window(?:EraseToPortBackground|Invalidate|NoOptions))))|e(?:c(?:3DES192|A(?:ES(?:1(?:28|92)|256)|ccountItemAttr|dd(?:Event(?:Mask)?|ressItemAttr)|lias|uthenticationType(?:Any|D(?:PA|efault)|HT(?:MLForm|TP(?:Basic|Digest))|ItemAttr|MSN|NTLM|RPA))|C(?:S(?:BasicValidateOnly|C(?:alculateCMSDigest|heck(?:AllArchitectures|GatekeeperArchitectures|NestedCode|TrustedAnchors)|on(?:siderExpiration|tentInformation))|D(?:e(?:dicatedHost|faultFlags)|oNotValidate(?:Executable|Resources)|ynamicInformation)|EnforceRevocationChecks|FullReport|GenerateGuestHash|InternalInformation|NoNetworkAccess|QuickCheck|Re(?:portProgress|quirementInformation|strict(?:S(?:idebandData|ymlinks)|ToAppLike))|S(?:i(?:gningInformation|ngleThreaded)|kipResourceDirectory|trictValidate)|Use(?:AllArchitectures|SoftwareSigningCert)|ValidatePEH)|ert(?:EncodingItemAttr|TypeItemAttr|ificate(?:Encoding|ItemClass|Type))|o(?:deS(?:ignature(?:Adhoc|Enforcement|Force(?:Expiration|Hard|Kill)|H(?:ashSHA(?:1|256(?:Truncated)?|384|512)|ost)|LibraryValidation|NoHash|R(?:estrict|untime))|tatus(?:Debugged|Hard|Kill|Platform|Valid))|mmentItemAttr)|r(?:e(?:at(?:ionDateItemAttr|orItemAttr)|dentialType(?:Default|NoUI|WithUI))|l(?:Encoding|Type))|ustomIconItemAttr)|De(?:fault(?:ChangedEvent(?:Mask)?|KeySize)|leteEvent(?:Mask)?|s(?:criptionItemAttr|ignatedRequirementType))|EveryEventMask|Format(?:BSAFE|NetscapeCertSequence|OpenSSL|P(?:EMSequence|KCS(?:12|7))|RawKey|SSH(?:v2)?|Unknown|Wrapped(?:LSH|OpenSSL|PKCS8|SSH)|X509Cert)|G(?:eneric(?:ItemAttr|PasswordItemClass)|uestRequirementType)|Ho(?:norRoot|stRequirementType)|I(?:n(?:ternetPasswordItemClass|v(?:alidRequirementType|isibleItemAttr))|ssuerItemAttr|tem(?:PemArmour|Type(?:Aggregate|Certificate|P(?:rivateKey|ublicKey)|SessionKey|Unknown)))|Key(?:A(?:l(?:ias|waysSensitive)|pplicationTag)|De(?:crypt|rive)|E(?:ffectiveKeySize|n(?:crypt|dDate)|xtractable)|ImportOnlyOne|Key(?:C(?:lass|reator)|SizeInBits|Type)|Label|Modifiable|N(?:everExtractable|oAccessControl)|P(?:ermanent|ri(?:ntName|vate))|S(?:e(?:curePassphrase|nsitive)|ign(?:Recover)?|tartDate)|U(?:nwrap|sage(?:All|C(?:RLSign|ontentCommitment|ritical)|D(?:ataEncipherment|ecipherOnly|igitalSignature)|EncipherOnly|Key(?:Agreement|CertSign|Encipherment)|NonRepudiation|Unspecified))|Verify(?:Recover)?|Wrap|chain(?:ListChanged(?:Event|Mask)|Prompt(?:Invalid(?:Act)?|RequirePassphase|Unsigned(?:Act)?)))|L(?:abelItemAttr|ibraryRequirementType|ockEvent(?:Mask)?)|M(?:atchBits|odDateItemAttr)|N(?:egativeItemAttr|oGuest)|OptionReserved|P(?:a(?:dding(?:None|OAEP|PKCS1(?:MD(?:2|5)|SHA(?:1|2(?:24|56)|384|512))?|SigRaw)|sswordChangedEvent(?:Mask)?|thItemAttr)|luginRequirementType|ortItemAttr|r(?:eferencesDomain(?:Common|Dynamic|System|User)|ivateKeyItemClass|otocol(?:ItemAttr|Type(?:A(?:FP|ny|ppleTalk)|C(?:IFS|VSpserver)|DAAP|EPPC|FTP(?:Account|Proxy|S)?|HTTP(?:Proxy|S(?:Proxy)?)?|I(?:MAP(?:S)?|PP|RC(?:S)?)|LDAP(?:S)?|NNTP(?:S)?|POP3(?:S)?|RTSP(?:Proxy)?|S(?:M(?:B|TP)|OCKS|SH|VN)|Telnet(?:S)?)))|ublicKey(?:HashItemAttr|ItemClass))|R(?:SAM(?:ax|in)|e(?:adPermStatus|quirementTypeCount|vocation(?:CRLMethod|NetworkAccessDisabled|OCSPMethod|PreferCRL|RequirePositiveResponse|UseAnyAvailableMethod)))|S(?:criptCodeItemAttr|e(?:curityDomainItemAttr|r(?:ialNumberItemAttr|v(?:erItemAttr|iceItemAttr)))|ignatureItemAttr|ubject(?:ItemAttr|KeyIdentifierItemAttr)|ymmetricKeyItemClass)|T(?:r(?:ansform(?:Error(?:A(?:bort(?:InProgress|ed)|ttributeNotFound)|Invalid(?:Algorithm|Connection|Input(?:Dictionary)?|Length|Operation|Type)|M(?:issingParameter|oreThanOneOutput)|N(?:ameAlreadyRegistered|otInitializedCorrectly)|UnsupportedAttribute)|Invalid(?:Argument|Override)|MetaAttribute(?:CanCycle|Deferred|Externalize|Has(?:InboundConnection|OutboundConnections)|Name|Re(?:f|quire(?:d|sOutboundConnection))|Stream|Value)|OperationNotSupportedOnGroup|TransformIs(?:Executing|NotRegistered))|ust(?:Option(?:AllowExpired(?:Root)?|FetchIssuerFromNet|ImplicitAnchors|LeafIsCA|RequireRevPerCert|UseTrustSettings)|Result(?:Deny|FatalTrustFailure|Invalid|OtherError|Proceed|RecoverableTrustFailure|Unspecified)|Settings(?:ChangedEvent(?:Mask)?|Domain(?:Admin|System|User)|KeyUse(?:Any|EnDecrypt(?:Data|Key)|KeyExchange|Sign(?:Cert|Revocation|ature))|Result(?:Deny|Invalid|Trust(?:AsRoot|Root)|Unspecified))))|ypeItemAttr)|U(?:nlock(?:Event(?:Mask)?|StateStatus)|pdateEvent(?:Mask)?|seOnly(?:GID|UID))|VolumeItemAttr|WritePermStatus|ondScale|p(?:192r1|256r1|384r1|521r1)|urityDomainKCItemAttr)|lector(?:All(?:1BitData|32BitData|4BitData|8BitData|AvailableData|HugeData|LargeData|MiniData|SmallData)|Huge(?:1Bit|32Bit|4Bit|8Bit(?:Mask)?)|Large(?:1Bit|32Bit|4Bit|8Bit(?:Mask)?)|Mini(?:1Bit|4Bit|8Bit)|Small(?:1Bit|32Bit|4Bit|8Bit(?:Mask)?)|sAre(?:Indexable|NotIndexable))|quenceTrackProperty_(?:AutomatedParameters|LoopInfo|MuteStatus|OffsetTime|SoloStatus|T(?:imeResolution|rackLength))|r(?:ialNumberKCItemAttr|v(?:erKCItemAttr|ice(?:KCItemAttr|sFolderType)))|t(?:CLUT(?:ByValue|Immediately|WithLuminance)|DebugOption|FrontProcess(?:CausedByUser|FrontWindowOnly)|PowerLevel))|h(?:a(?:dowDialogVariantCode|r(?:ed(?:BadgeIcon|Folder(?:AliasType|Icon(?:Resource)?)|LibrariesFolder(?:Icon|Type)|UserDataFolderType)|ingPrivs(?:NotApplicableIcon|Read(?:OnlyIcon|WriteIcon)|UnknownIcon|WritableIcon)))|eet(?:AlertWindowClass|WindowClass)|ift(?:JIS_(?:BasicVariant|DOSVariant|MusicCDVariant)|Unicode)|o(?:rtcutIcon|w(?:DiacriticsSelector|HideInputWindow))|utdown(?:FolderType|Items(?:DisabledFolder(?:Icon|Type)|FolderIcon)))|i(?:deFloaterVariantCode|gn(?:KCItemAttr|atureKCItemAttr)|mpl(?:eWindowClass|ifiedCharactersSelector))|l(?:ash(?:ToDivideO(?:ffSelector|nSelector)|edZeroO(?:ffSelector|nSelector))|eep(?:De(?:mand|ny)|Now|Re(?:quest|voke)|Unlock|WakeUp))|ma(?:ll(?:1BitMask|32BitData|4Bit(?:Data|IconSize)|8Bit(?:Data|IconSize|Mask)|CapsSelector|IconSize)|rt(?:QuotesO(?:ffSelector|nSelector)|SwashType))|o(?:rt(?:AscendingIcon|DescendingIcon)|und(?:FileIcon|SetsFolderType))|p(?:a(?:ceCharCode|tial(?:Mixer(?:AttenuationCurve_(?:Exponential|Inverse|Linear|Power)|Param_(?:Azimuth|Distance|E(?:levation|nable)|G(?:ain|lobalReverbGain)|M(?:axGain|inGain)|O(?:bstructionAttenuation|cclusionAttenuation)|PlaybackRate|ReverbBlend)|RenderingFlags_(?:DistanceAttenuation|InterAuralDelay))|izationAlgorithm_(?:EqualPowerPanning|HRTF(?:HQ)?|S(?:oundField|phericalHead|tereoPassThrough)|VectorBasedPanning)))|e(?:ak(?:ableItemsFolder(?:Type)?|erConfiguration_(?:5_(?:0|1)|HeadPhones|Quad|Stereo))|cial(?:Case(?:CaretHook|DrawHook|EOLHook|GNEFilterProc|Hi(?:ghHook|tTestHook)|MBarHook|NWidthHook|ProtocolHandler|S(?:elector(?:Mask|Phase|Width)|ocketListener)|T(?:E(?:DoText|FindWord|Recalc)|extWidthHook)|WidthHook)?|Folder)|ech(?:FolderType|GenerateTune|InputMethodClass|Relative(?:Duration|Pitch)|ShowSyllables))|otlight(?:ImportersFolderType|MetadataCacheFolderType|SavedSearchesFolderType))|quaredLigaturesO(?:ffSelector|nSelector)|t(?:a(?:ck(?:DispatchedPascalStackBased|OverflowException|Parameter(?:Mask|Phase|Width))|ndardWindowDefinition|rt(?:DateKCItemAttr|up(?:Folder(?:AliasType|IconResource|Type)|Items(?:DisabledFolder(?:Icon|Type)|FolderIcon)))|ti(?:cTextDialogItem|oneryFolderType))|d(?:AlertDoNot(?:AnimateOn(?:Cancel|Default|Other)|CloseOnHelp|DisposeSheet)|C(?:FStringAlertVersion(?:One|Two)|ancelItemIndex)|OkItemIndex)|ereoMixerParam_(?:P(?:an|ost(?:AveragePower|PeakHoldLevel)|re(?:AveragePower|PeakHoldLevel))|Volume)|illIdle|o(?:p(?:Icon|pedThreadState)|red(?:BasicWindowDescriptionID|Window(?:PascalTitleID|SystemTag|TitleCFStringID)))|yl(?:eOptionsType|isticAlt(?:E(?:ight(?:O(?:ffSelector|nSelector)|eenO(?:ffSelector|nSelector))|levenO(?:ffSelector|nSelector))|F(?:i(?:fteenO(?:ffSelector|nSelector)|veO(?:ffSelector|nSelector))|our(?:O(?:ffSelector|nSelector)|teenO(?:ffSelector|nSelector)))|Nine(?:O(?:ffSelector|nSelector)|teenO(?:ffSelector|nSelector))|OneO(?:ffSelector|nSelector)|S(?:even(?:O(?:ffSelector|nSelector)|teenO(?:ffSelector|nSelector))|ix(?:O(?:ffSelector|nSelector)|teenO(?:ffSelector|nSelector)))|T(?:enO(?:ffSelector|nSelector)|h(?:irteenO(?:ffSelector|nSelector)|reeO(?:ffSelector|nSelector))|w(?:e(?:lveO(?:ffSelector|nSelector)|ntyO(?:ffSelector|nSelector))|oO(?:ffSelector|nSelector)))|ernativesType)))|u(?:b(?:jectKCItemAttr|stituteVerticalFormsO(?:ffSelector|nSelector))|p(?:eriorsSelector|ports(?:FileTranslation|ScrapTranslation))|spend(?:Demand|Re(?:quest|voke)|Wake(?:ToDoze|Up)))|washAlternatesO(?:ffSelector|nSelector)|y(?:m(?:Link(?:Creator|FileType)|bolLigaturesO(?:ffSelector|nSelector))|s(?:SWTooOld|tem(?:ControlPanelFolderType|D(?:esktopFolderType|omain)|E(?:ventKCEventMask|xtensionDisabledFolder(?:Icon|Type))|Folder(?:AliasType|Icon(?:Resource)?|Type)|IconsCreator|KCEvent|P(?:PDDomain|r(?:eferencesFolderType|ocess))|ResFile|S(?:ound(?:ID_(?:FlashScreen|UserPreferredAlert|Vibrate)|sFolderType)|uitcaseIcon)|TrashFolderType))))|T(?:EC(?:A(?:dd(?:F(?:allbackInterrupt(?:Bit|Mask)|orceASCIIChanges(?:Bit|Mask))|TextRunHeuristics(?:Bit|Mask))|rrayFullErr|vailable(?:EncodingsResType|SniffersResType))|B(?:adTextRunErr|ufferBelowMinimumSizeErr)|C(?:hinesePluginSignature|o(?:nversionInfoResType|rruptConverterErr))|Di(?:rectionErr|sable(?:Fallbacks(?:Bit|Mask)|LooseMappings(?:Bit|Mask)))|FallbackTextLengthFix(?:Bit|Mask)|GlobalsUnavailableErr|I(?:n(?:completeElementErr|foCurrentFormat|ternetName(?:DefaultUsageMask|StrictUsageMask|TolerantUsageMask|sResType))|temUnavailableErr)|JapanesePluginSignature|K(?:eepInfoFix(?:Bit|Mask)|oreanPluginSignature)|M(?:ailEncodingsResType|issingTableErr)|N(?:eedFlushStatus|oConversionPathErr)|OutputBufferFullStatus|P(?:artialCharErr|lugin(?:Creator|DispatchTable(?:CurrentVersion|Version1(?:_(?:1|2))?)|ManyToOne|OneTo(?:Many|One)|SniffObj|Type)|referredEncodingFix(?:Bit|Mask))|ResourceID|S(?:ignature|ubTextEncodingsResType)|T(?:able(?:ChecksumErr|FormatErr)|ext(?:RunBitClearFix(?:Bit|Mask)|ToUnicodeScanFix(?:Bit|Mask)))|U(?:n(?:icodePluginSignature|mappableElementErr)|sedFallbacksStatus)|WebEncodingsResType|_MIBEnumDontCare)|MTaskActive|RAK(?:CurrentVersion|Tag|UniformFormat)|SM(?:15Version|2(?:0Version|2Version|3Version|4Version)|Doc(?:Access(?:EffectiveRangeAttribute(?:Bit)?|FontSizeAttribute(?:Bit)?)|ument(?:EnabledInputSourcesPropertyTag|Input(?:ModePropertyTag|SourceOverridePropertyTag)|Property(?:SupportGlyphInfo|UnicodeInputWindow)|RefconPropertyTag|Support(?:DocumentAccessPropertyTag|GlyphInfoPropertyTag)|T(?:SMTEPropertyTag|extServicePropertyTag)|U(?:nicode(?:InputWindowPropertyTag|PropertyTag)|seFloatingWindowPropertyTag)|WindowLevelPropertyTag))|Hilite(?:BlockFillText|C(?:aretPosition|onvertedText)|NoHilite|OutlineText|RawText|Selected(?:ConvertedText|RawText|Text))|InsideOf(?:ActiveInputArea|Body)|OutsideOfBody|TEDocumentInterfaceType|Version)|XN(?:A(?:IFFFile|TSUI(?:Font(?:FeaturesAttribute|VariationsAttribute)|IsNotInstalledErr|Style(?:Continuous(?:Bit|Mask)|Size)?)|l(?:ign(?:CenterAction|LeftAction|RightAction)|lCountMask|readyInitializedErr|waysWrapAtViewEdge(?:Bit|Mask))|ttributeTagInvalidForRunErr|uto(?:Indent(?:O(?:ff|n)|StateTag)|Scroll(?:BehaviorTag|InsertionIntoView|Never|WhenInsertionVisible)|Wrap))|Ba(?:ckgroundTypeRGB|dDefaultFileTypeWarning)|C(?:annot(?:AddFrameErr|SetAutoIndentErr|TurnTSMOffWhenUsingUnicodeErr)|enter(?:Tab)?|hange(?:Font(?:Action|ColorAction|SizeAction)|StyleAction)|lear(?:Action|Th(?:eseFontFeatures|isControl))|o(?:lorContinuous(?:Bit|Mask)|pyNotAllowedInEchoModeErr)|utAction)|D(?:ataTypeNotAllowedErr|e(?:crementTypeSize|stinationRectKey)|isable(?:DragAndDrop(?:Bit|Mask|Tag)?|LayoutAndDraw(?:Tag)?|dFunctionalityErr)|o(?:FontSubstitution(?:Bit|Mask)?|NotInstallDragProcs(?:Bit|Mask)|nt(?:CareTypeS(?:ize|tyle)|Draw(?:CaretWhenInactive(?:Bit|Mask)?|SelectionWhenInactive(?:Bit|Mask)?)))|r(?:aw(?:CaretWhenInactive(?:Tag)?|GrowIcon(?:Bit|Mask)|Item(?:AllMask|Scrollbars(?:Bit|Mask)|Text(?:AndSelection(?:Bit|Mask)|Bit|Mask))|SelectionWhenInactive(?:Tag)?)|opAction))|En(?:able(?:DragAndDrop|LayoutAndDraw)|d(?:IterationErr|Offset)|tireWord(?:Bit|Mask))|F(?:l(?:attenMoviesTag|ush(?:Default|Left|Right))|o(?:nt(?:Continuous(?:Bit|Mask)|FeatureAction|SizeAttributeSize|VariationAction)|rceFullJust)|ullJust)|Horizontal(?:ScrollBarRectKey)?|I(?:OPrivilegesTag|gnoreCase(?:Bit|Mask)|llegalToCrossDataBoundariesErr|mageWithQD(?:Bit|Mask)|n(?:crementTypeSize|lineStateTag|valid(?:FrameIDErr|RunIndex)))|JustificationTag|L(?:eftT(?:ab|oRight)|in(?:eDirectionTag|k(?:NotPressed|Tracking|WasPressed)))|M(?:a(?:cOSEncoding|rginsTag)|o(?:nostyledText(?:Bit|Mask)|veAction)|ultiple(?:FrameType|StylesPerTextDocumentResType))|No(?:A(?:ppleEventHandlers(?:Bit|Mask)|utoWrap)|FontVariations|MatchErr|Selection(?:Bit|Mask)|TSMEver(?:Bit|Mask)|UserIOTag)|O(?:perationNotAllowedErr|utsideOf(?:FrameErr|LineErr))|Pa(?:geFrameType|steAction)|QDFont(?:ColorAttribute(?:Size)?|FamilyIDAttribute(?:Size)?|NameAttribute(?:Size)?|S(?:izeAttribute(?:Size)?|tyleAttribute(?:Size)?))|R(?:e(?:ad(?:Only(?:Bit|Mask)?|Write)|fConTag|startAppleEventHandlers(?:Bit|Mask))|i(?:chTextFormatData|ghtT(?:ab|oLeft))|un(?:Count(?:Bit|Mask)|IndexOutofBoundsErr))|S(?:aveStylesAsSTYLResource(?:Bit|Mask)|crollUnitsIn(?:Lines|Pixels|ViewRects)|election(?:O(?:ff|n)|StateTag)|how(?:End|Start|Window(?:Bit|Mask))|i(?:ngle(?:L(?:evelUndoTag|ineOnly(?:Bit|Mask))|StylePerTextDocumentResType)|zeContinuous(?:Bit|Mask))|omeOrAllTagsInvalidForRunErr|t(?:artOffset|yleContinuous(?:Bit|Mask))|upport(?:EditCommand(?:Processing|Updating)|FontCommand(?:Processing|Updating)|SpellCheckCommand(?:Processing|Updating))|ystemDefaultEncoding)|T(?:abSettingsTag|ext(?:Data|E(?:ditStyleFrameType|ncodingAttribute(?:Size)?)|File|InputCount(?:Bit|Mask)|RectKey|ensionFile)|ypingAction)|U(?:RLAttribute|n(?:doLastAction|icode(?:Encoding|Text(?:Data|File)))|se(?:Bottomline|C(?:arbonEvents|urrentSelection)|EncodingWordRules(?:Bit|Mask)|Inline|QDforImaging(?:Bit|Mask)|ScriptDefaultValue|rCanceledOperationErr))|V(?:ertical(?:ScrollBarRectKey)?|i(?:ewRectKey|sibilityTag))|W(?:ant(?:HScrollBar(?:Bit|Mask)|VScrollBar(?:Bit|Mask))|illDefaultTo(?:ATSUI(?:Bit|Mask)|CarbonEvent(?:Bit|Mask))|ordWrapStateTag))|a(?:bCharCode|llCapsSelector|sk(?:CreationException|TerminationException))|e(?:mporary(?:FolderType|ItemsIn(?:CacheDataFolderType|UserDomainFolderType))|xt(?:Center|Encoding(?:ANSEL|B(?:aseName|ig5(?:_(?:E|HKSCS_1999))?)|CNS_11643_92_P(?:1|2|3)|D(?:OS(?:Arabic|BalticRim|C(?:anadianFrench|hinese(?:Simplif|Trad)|yrillic)|Greek(?:1|2)?|Hebrew|Icelandic|Japanese|Korean|Latin(?:1|2|US)|Nordic|Portuguese|Russian|T(?:hai|urkish))|efault(?:Format|Variant))|E(?:BCDIC_(?:CP037|LatinCore|US)|UC_(?:CN|JP|KR|TW))|F(?:ormatName|ullName)|GB(?:K_95|_(?:18030_200(?:0|5)|2312_80))|HZ_GB_2312|ISO(?:10646_1993|Latin(?:1(?:0)?|2|3|4|5|6|7|8|9|Arabic|Cyrillic|Greek|Hebrew)|_2022_(?:CN(?:_EXT)?|JP(?:_(?:1|2|3))?|KR))|JIS_(?:C6226_78|X02(?:0(?:1_76|8_(?:83|90))|1(?:2_90|3_MenKuTen)))|K(?:OI8_(?:R|U)|SC_5601_(?:87|92_Johab))|M(?:ac(?:Ar(?:abic|menian)|B(?:engali|urmese)|C(?:e(?:ltic|ntralEurRoman)|hinese(?:Simp|Trad)|roatian|yrillic)|D(?:evanagari|ingbats)|E(?:astEurRoman|thiopic|xtArabic)|Farsi|G(?:aelic|e(?:ez|orgian)|reek|u(?:jarati|rmukhi))|H(?:FS|ebrew)|I(?:celandic|nuit)|Japanese|K(?:annada|eyboardGlyphs|hmer|orean)|Laotian|M(?:alayalam|ongolian)|Oriya|R(?:Symbol|oman(?:Latin1|ian)?)|S(?:i(?:mpChinese|nhalese)|ymbol)|T(?:amil|elugu|hai|ibetan|radChinese|urkish)|U(?:krainian|ni(?:code|nterp))|V(?:T100|ietnamese))|ultiRun)|NextStep(?:Japanese|Latin)|ShiftJIS(?:_X0213(?:_00)?)?|U(?:S_ASCII|n(?:icode(?:Default|V(?:1(?:0_0|1_0|2_1|_1)|2_(?:0|1)|3_(?:0|1|2)|4_0|5_(?:0|1)|6_(?:0|1|3)|7_0|8_0|9_0))|known))|V(?:ISCII|ariantName)|Windows(?:A(?:NSI|rabic)|BalticRim|Cyrillic|Greek|Hebrew|KoreanJohab|Latin(?:1|2|5)|Vietnamese)|sFolder(?:Icon|Type))|Flush(?:Default|Left|Right)|LanguageDontCare|MalformedInputErr|RegionDontCare|S(?:criptDontCare|ervice(?:Class|DocumentInterfaceType|InputModePropertyTag|JaTypingMethodPropertyTag)?|pacingType)|ToSpeech(?:SynthType|Voice(?:BundleType|FileType|Type))|Un(?:definedElementErr|supportedEncodingErr)))|h(?:eme(?:A(?:ctive(?:Alert(?:BackgroundBrush|TextColor)|BevelButtonTextColor|D(?:ialog(?:BackgroundBrush|TextColor)|ocumentWindowTitleTextColor)|M(?:enuItemTextColor|o(?:delessDialog(?:BackgroundBrush|TextColor)|vableModalWindowTitleTextColor))|P(?:lacardTextColor|opup(?:ArrowBrush|ButtonTextColor|LabelTextColor|WindowTitleColor)|ushButtonTextColor)|RootMenuTextColor|ScrollBarDelimiterBrush|UtilityWindow(?:BackgroundBrush|TitleTextColor)|WindowHeaderTextColor)|dornment(?:Arrow(?:Do(?:ubleArrow|wnArrow)|LeftArrow|RightArrow|UpArrow)|D(?:efault|rawIndicatorOnly)|Focus|Header(?:Button(?:LeftNeighborSelected|NoS(?:hadow|ortArrow)|RightNeighborSelected|S(?:hadowOnly|ortUp))|MenuButton)|No(?:Shadow|ne)|RightToLeft|ShadowOnly)|l(?:ert(?:HeaderFont|Window)|iasArrowCursor)|pp(?:earanceFileNameTag|l(?:eGuideCoachmarkBrush|icationFont))|rrow(?:3pt|5pt|7pt|9pt|Button(?:Mini|Small)?|Cursor|Down|Left|Right|Up))|B(?:ackground(?:ListViewWindowHeader|Metal|Placard|SecondaryGroupBox|TabPane|WindowHeader)|evelButton(?:Inset|Large|Medium|Small)?|ottom(?:InsideArrowPressed|OutsideArrowPressed|TrackPressed)|rush(?:A(?:ctiveAreaFill|l(?:ertBackground(?:Active|Inactive)|ternatePrimaryHighlightColor)|ppleGuideCoachmark)|B(?:evel(?:Active(?:Dark|Light)|Inactive(?:Dark|Light))|lack|utton(?:Active(?:Dark(?:Highlight|Shadow)|Light(?:Highlight|Shadow))|F(?:ace(?:Active|Inactive|Pressed)|rame(?:Active|Inactive))|Inactive(?:Dark(?:Highlight|Shadow)|Light(?:Highlight|Shadow))|Pressed(?:Dark(?:Highlight|Shadow)|Light(?:Highlight|Shadow))))|ChasingArrows|D(?:ialogBackground(?:Active|Inactive)|ocumentWindowBackground|ra(?:gHilite|werBackground))|F(?:inderWindowBackground|ocusHighlight)|IconLabelBackground(?:Selected)?|ListView(?:Background|ColumnDivider|EvenRowBackground|OddRowBackground|S(?:eparator|ortColumnBackground))|M(?:enuBackground(?:Selected)?|o(?:delessDialogBackground(?:Active|Inactive)|vableModalBackground))|NotificationWindowBackground|P(?:assiveAreaFill|opupArrow(?:Active|Inactive|Pressed)|rimaryHighlightColor)|S(?:crollBarDelimiter(?:Active|Inactive)|econdaryHighlightColor|heetBackground(?:Opaque|Transparent)?|taticAreaFill)|ToolbarBackground|UtilityWindowBackground(?:Active|Inactive)|White)|utton(?:Mixed|O(?:ff|n)))|C(?:h(?:asingArrowsBrush|eckBox(?:C(?:heckMark|lassicX)|Mini|Small)?)|losedHandCursor|o(?:mboBox(?:Mini|Small)?|nt(?:extualMenuArrowCursor|rolSoundsMask)|pyArrowCursor|unting(?:DownHandCursor|Up(?:AndDownHandCursor|HandCursor)))|rossCursor|u(?:rrentPortFont|stomThemesFileType))|D(?:ataFileType|blClickCollapseTag|e(?:faultAdornment|sktopP(?:attern(?:NameTag|Tag)|icture(?:Ali(?:asTag|gnmentTag)|NameTag)))|i(?:alogWindow|s(?:abled(?:MenuItemTextColor|RootMenuTextColor)|closure(?:Button|Down|Left|Right|Triangle)))|ocumentWindow(?:BackgroundBrush)?|ra(?:g(?:HiliteBrush|Sound(?:Dragging|Grow(?:UtilWindow|Window)|Move(?:Alert|Dialog|Icon|UtilWindow|Window)|None|S(?:crollBar(?:Arrow(?:Decreasing|Increasing)|Ghost|Thumb)|lider(?:Ghost|Thumb))))|w(?:IndicatorOnly|erWindow)))|E(?:mphasizedSystemFont|xamplePictureIDTag)|F(?:inder(?:SoundsMask|WindowBackgroundBrush)|ocus(?:Adornment|HighlightBrush))|Grow(?:Down|Left|Right|Up)|HighlightColor(?:NameTag|Tag)|I(?:BeamCursor|conLabel(?:BackgroundBrush|TextColor)|n(?:active(?:Alert(?:BackgroundBrush|TextColor)|BevelButtonTextColor|D(?:ialog(?:BackgroundBrush|TextColor)|ocumentWindowTitleTextColor)|Mo(?:delessDialog(?:BackgroundBrush|TextColor)|vableModalWindowTitleTextColor)|P(?:lacardTextColor|opup(?:ArrowBrush|ButtonTextColor|LabelTextColor|WindowTitleColor)|ushButtonTextColor)|ScrollBarDelimiterBrush|UtilityWindow(?:BackgroundBrush|TitleTextColor)|WindowHeaderTextColor)|cDecButton(?:Mini|Small)?|determinateBar(?:Large|M(?:edium|ini))?))|L(?:a(?:belFont|rge(?:BevelButton|IndeterminateBar|ProgressBar|RoundButton|TabHeight(?:Max)?))|eft(?:InsideArrowPressed|OutsideArrowPressed|TrackPressed)|ist(?:HeaderButton|View(?:BackgroundBrush|S(?:eparatorBrush|ortColumnBackgroundBrush)|TextColor)))|M(?:e(?:dium(?:BevelButton|IndeterminateBar|ProgressBar|S(?:crollBar|lider))|nu(?:Active|Bar(?:Inactive|Normal|Selected)|Disabled|Item(?:A(?:lignRight|t(?:Bottom|Top))|CmdKeyFont|Font|H(?:asIcon|ier(?:Background|archical))|MarkFont|NoBackground|P(?:lain|opUpBackground)|Scroll(?:DownArrow|UpArrow))|S(?:elected|oundsMask|quareMenuBar)|T(?:itleFont|ype(?:Hierarchical|Inactive|P(?:opUp|ullDown))))|tric(?:B(?:estListHeaderHeight|uttonRounded(?:Height|RecessedHeight))|C(?:heckBox(?:GlyphHeight|Height|Width)|omboBox(?:Large(?:BottomShadowOffset|DisclosureWidth|RightShadowOffset)|Mini(?:BottomShadowOffset|DisclosureWidth|RightShadowOffset)|Small(?:BottomShadowOffset|DisclosureWidth|RightShadowOffset)))|Disclosure(?:Button(?:Height|Size|Width)|Triangle(?:Height|Width))|EditText(?:FrameOutset|Whitespace)|FocusRectOutset|HSlider(?:Height|Tick(?:Height|Offset))|ImageWellThickness|L(?:arge(?:ProgressBarThickness|RoundButtonSize|Tab(?:CapsWidth|Height))|i(?:st(?:BoxFrameOutset|HeaderHeight)|ttleArrows(?:Height|Mini(?:Height|Width)|Small(?:Height|Width)|Width)))|M(?:enu(?:ExcludedMarkColumnWidth|I(?:conTrailingEdgeMargin|ndentWidth)|Mark(?:ColumnWidth|Indent)|Text(?:LeadingEdgeMargin|TrailingEdgeMargin))|ini(?:CheckBox(?:Height|Width)|DisclosureButton(?:Height|Width)|HSlider(?:Height|MinThumbWidth|Tick(?:Height|Offset))|P(?:opupButtonHeight|u(?:llDownHeight|shButtonHeight))|RadioButton(?:Height|Width)|Tab(?:CapsWidth|FrameOverlap|Height|Overlap)|VSlider(?:MinThumbHeight|Tick(?:Offset|Width)|Width)))|NormalProgressBarThickness|P(?:aneSplitterHeight|opupButtonHeight|r(?:imaryGroupBoxContentInset|ogressBar(?:ShadowOutset|Thickness))|u(?:llDownHeight|shButtonHeight))|R(?:adioButton(?:GlyphHeight|Height|Width)|e(?:levanceIndicatorHeight|sizeControlHeight)|ound(?:ButtonSize|TextField(?:Content(?:Height|Inset(?:Bottom|Left|Right|Top|WithIcon(?:Left|Right)))|MiniContent(?:Height|Inset(?:Bottom|Left|Right|Top|WithIcon(?:Left|Right)))|SmallContent(?:Height|Inset(?:Bottom|Left|Right|Top|WithIcon(?:Left|Right))))))|S(?:crollBar(?:MinThumb(?:Height|Width)|Overlap|Width)|e(?:condaryGroupBoxContentInset|paratorSize)|liderMinThumb(?:Height|Width)|mall(?:CheckBox(?:Height|Width)|DisclosureButton(?:Height|Width)|HSlider(?:Height|MinThumbWidth|Tick(?:Height|Offset))|P(?:aneSplitterHeight|opupButtonHeight|rogressBar(?:ShadowOutset|Thickness)|u(?:llDownHeight|shButtonHeight))|R(?:adioButton(?:Height|Width)|esizeControlHeight)|ScrollBar(?:MinThumb(?:Height|Width)|Width)|Tab(?:CapsWidth|FrameOverlap|Height|Overlap)|VSlider(?:MinThumbHeight|Tick(?:Offset|Width)|Width)))|T(?:ab(?:FrameOverlap|IndentOrStyle|Overlap)|extured(?:PushButtonHeight|SmallPushButtonHeight)|itleBarControlsHeight)|VSlider(?:Tick(?:Offset|Width)|Width)))|ini(?:CheckBox|IndeterminateBar|ProgressBar|RadioButton|S(?:crollBar|lider|ystemFont))|ovable(?:AlertWindow|DialogWindow))|N(?:ameTag|o(?:Adornment|Sounds|rmal(?:CheckBox|RadioButton)|tAllowedCursor))|OpenHandCursor|P(?:l(?:a(?:inDialogWindow|tinumFileType)|usCursor)|o(?:intingHandCursor|ofCursor|pup(?:Button(?:Mini|Normal|Small)?|Tab(?:CenterOn(?:Offset|Window)|NormalPosition)|Window))|r(?:essed(?:BevelButtonTextColor|P(?:lacardTextColor|opup(?:ArrowBrush|ButtonTextColor)|ushButtonTextColor))|ogressBar(?:Large|M(?:edium|ini))?)|ushButton(?:Font|Inset(?:Small)?|Mini|Normal|Small|Textured(?:Small)?)?)|R(?:adioButton(?:Mini|Small)?|e(?:levanceBar|size(?:DownCursor|Left(?:Cursor|RightCursor)|RightCursor|Up(?:Cursor|DownCursor)))|ight(?:InsideArrowPressed|OutsideArrowPressed|T(?:oLeftAdornment|rackPressed))|ound(?:Button(?:Help|Large)?|edBevelButton))|S(?:avvyMenuResponse|crollBar(?:Arrow(?:StyleTag|s(?:LowerRight|Single))|M(?:edium|ini)|Small|Thumb(?:Normal|Proportional|StyleTag))?|elected(?:MenuItemTextColor|RootMenuTextColor)|h(?:adowDialogWindow|eetWindow)|lider(?:M(?:edium|ini)|Small)?|m(?:all(?:BevelButton|CheckBox|EmphasizedSystemFont|RadioButton|S(?:crollBar|lider|ystemFont(?:Tag)?)|TabHeight(?:Max)?)|oothFont(?:EnabledTag|MinSizeTag))|ound(?:Alert(?:Close|Open)|B(?:alloon(?:Close|Open)|evel(?:E(?:nter|xit)|Press|Release)|utton(?:E(?:nter|xit)|Press|Release))|C(?:ancelButton(?:E(?:nter|xit)|Press|Release)|heckbox(?:E(?:nter|xit)|Press|Release)|opyDone)|D(?:efaultButton(?:E(?:nter|xit)|Press|Release)|i(?:alog(?:Close|Open)|s(?:closure(?:E(?:nter|xit)|Press|Release)|k(?:Eject|Insert)))|ragTarget(?:Drop|Hilite|Unhilite))|EmptyTrash|FinderDragO(?:ffIcon|nIcon)|L(?:aunchApp|ittleArrow(?:Dn(?:Press|Release)|E(?:nter|xit)|Up(?:Press|Release)))|M(?:askTag|enu(?:Close|Item(?:Hilite|Release)|Open))|N(?:ewItem|one)|Popup(?:E(?:nter|xit)|Press|Release|Window(?:Close|Open))|R(?:adio(?:E(?:nter|xit)|Press|Release)|e(?:ceiveDrop|solveAlias))|S(?:croll(?:Arrow(?:E(?:nter|xit)|Press|Release)|EndOfTrack|TrackPress)|electItem|lider(?:EndOfTrack|TrackPress))|T(?:ab(?:E(?:nter|xit)|Pressed|Release)|rack(?:FileType|NameTag))|UtilWin(?:C(?:lose(?:E(?:nter|xit)|Press|Release)|ollapse(?:E(?:nter|xit)|Press|Release))|DragBoundary|Zoom(?:E(?:nter|xit)|Press|Release)|dow(?:Activate|C(?:lose|ollapse(?:Down|Up))|Open|Zoom(?:In|Out)))|Window(?:Activate|C(?:lose(?:E(?:nter|xit)|Press|Release)?|ollapse(?:Down|E(?:nter|xit)|Press|Release|Up))|DragBoundary|Open|Zoom(?:E(?:nter|xit)|In|Out|Press|Release))|sEnabledTag)|p(?:ecifiedFont|inningCursor)|tate(?:Active|Disabled|Inactive|Pressed(?:Down|Up)?|Rollover|Unavailable(?:Inactive)?)|ystemFont(?:Detail(?:Emphasized)?|Tag)?)|T(?:ab(?:East|Front(?:Inactive|Unavailable)?|No(?:nFront(?:Inactive|Pressed|Unavailable)?|rth)|PaneOverlap|South|West)|extColor(?:Alert(?:Active|Inactive)|B(?:evelButton(?:Active|Inactive|Pressed|Sticky(?:Active|Inactive))|lack)|D(?:ialog(?:Active|Inactive)|ocumentWindowTitle(?:Active|Inactive))|IconLabel(?:Selected)?|ListView|M(?:enuItem(?:Active|Disabled|Selected)|o(?:delessDialog(?:Active|Inactive)|vableModalWindowTitle(?:Active|Inactive)))|Notification|P(?:lacard(?:Active|Inactive|Pressed)|opup(?:Button(?:Active|Inactive|Pressed)|Label(?:Active|Inactive)|WindowTitle(?:Active|Inactive))|ushButton(?:Active|Inactive|Pressed))|RootMenu(?:Active|Disabled|Selected)|SystemDetail|Tab(?:Front(?:Active|Inactive)|NonFront(?:Active|Inactive|Pressed))|UtilityWindowTitle(?:Active|Inactive)|W(?:hite|indowHeader(?:Active|Inactive)))|humb(?:Downward|P(?:lain|ressed)|Upward)|o(?:olbarFont|p(?:InsideArrowPressed|OutsideArrowPressed|TrackPressed))|rack(?:Active|Disabled|H(?:asFocus|ideTrack|orizontal)|Inactive|No(?:ScrollBarArrows|thingToScroll)|RightToLeft|ShowThumb|ThumbRgnIsNotGhost))|U(?:serDefinedTag|tility(?:SideWindow|Window(?:TitleFont)?))|V(?:ariant(?:BaseTintTag|NameTag)|iewsFont(?:SizeTag|Tag)?)|W(?:atchCursor|i(?:dget(?:C(?:loseBox|ollapseBox)|DirtyCloseBox|ToolbarButton|ZoomBox)|ndow(?:Has(?:C(?:loseBox|ollapseBox)|Dirty|FullZoom|Grow|HorizontalZoom|T(?:itleText|oolbarButton)|VerticalZoom)|IsCollapsed|SoundsMask|TitleFont)))|sFolderType)|i(?:nkCStackBased|rdWidth(?:NumbersSelector|TextSelector))|umbnail(?:32BitData|8BitMask))|i(?:ckScale|le(?:IconVariant|dOnScreen)|mePitchParam_(?:EffectBlend|Pitch|Rate)|tlingCapsSelector)|oo(?:ManyIOWindowsErr|lbar(?:A(?:dvancedIcon|pplicationsFolderIcon)|CustomizeIcon|D(?:e(?:leteIcon|sktopFolderIcon)|o(?:cumentsFolderIcon|wnloadsFolderIcon))|FavoritesIcon|HomeIcon|InfoIcon|L(?:abelsIcon|ibraryFolderIcon)|M(?:ovieFolderIcon|usicFolderIcon)|P(?:icturesFolderIcon|ublicFolderIcon)|SitesFolderIcon|UtilitiesFolderIcon|WindowClass))|r(?:a(?:c(?:eException|kMouseLocationOption(?:DontConsumeMouseUp|IncludeScrollWheel))|ditional(?:Alt(?:F(?:iveSelector|ourSelector)|OneSelector|T(?:hreeSelector|woSelector))|CharactersSelector|NamesCharactersSelector)|ns(?:codingCompositionO(?:ffSelector|nSelector)|form(?:Disabled|Label(?:1|2|3|4|5|6|7)|None|O(?:ffline|pen)|Selected(?:Disabled|O(?:ffline|pen))?)|l(?:at(?:e(?:Get(?:FileTranslationList|ScrapTranslationList(?:ConsideringData)?|TranslatedFilename)|Identify(?:File|Scrap)|Translate(?:File|Scrap))|ion(?:DataTranslation|FileTranslation|ScrapProgressDialogID)|orCanGenerateFilename)|iterationType)|parentEncod(?:edPixel|ing(?:Shift)?))|pException|sh(?:FolderType|Icon(?:Resource)?))|ueType(?:F(?:latFontIcon|ontIcon)|MultiFlatFontIcon)|yAuthenticate)|wo(?:ByteCode|WayEncryptPassword)|yp(?:eKCItemAttr|ographicExtrasType))|U(?:AZoomFocusType(?:InsertionPoint|Other)|C(?:BidiCat(?:ArabicNumber|B(?:lockSeparator|oundaryNeutral)|CommonNumberSeparator|EuroNumber(?:Separator|Terminator)?|FirstStrongIsolate|LeftRight(?:Embedding|Isolate|Override)?|No(?:nSpacingMark|tApplicable)|OtherNeutral|PopDirectional(?:Format|Isolate)|RightLeft(?:Arabic|Embedding|Isolate|Override)?|SegmentSeparator|Whitespace)|C(?:harPropType(?:BidiCategory|CombiningClass|DecimalDigitValue|GenlCategory)|ollate(?:C(?:aseInsensitiveMask|omposeInsensitiveMask)|Di(?:acritInsensitiveMask|gits(?:AsNumberMask|OverrideMask))|PunctuationSignificantMask|StandardOptions|Type(?:HFSExtended|Mask|S(?:hiftBits|ourceMask))|WidthInsensitiveMask))|GenlCat(?:Letter(?:Lowercase|Modifier|Other|Titlecase|Uppercase)|Mark(?:Enclosing|NonSpacing|SpacingCombining)|Number(?:DecimalDigit|Letter|Other)|Other(?:Control|Format|NotAssigned|PrivateUse|Surrogate)|Punct(?:C(?:lose|onnector)|Dash|FinalQuote|InitialQuote|O(?:pen|ther))|S(?:eparator(?:Line|Paragraph|Space)|ymbol(?:Currency|M(?:ath|odifier)|Other)))|HighSurrogateRange(?:End|Start)|Key(?:Action(?:AutoKey|D(?:isplay|own)|Up)|Layout(?:FeatureInfoFormat|HeaderFormat)|ModifiersToTableNumFormat|Output(?:GetIndexMask|S(?:equenceIndexMask|tateIndexMask)|TestForIndexMask)|S(?:equenceDataIndexFormat|tate(?:Entry(?:RangeFormat|TerminalFormat)|RecordsIndexFormat|TerminatorsFormat))|T(?:oCharTableIndexFormat|ranslateNoDeadKeys(?:Bit|Mask)))|LowSurrogateRange(?:End|Start)|OutputBufferTooSmall|T(?:S(?:Direction(?:Next|Previous)|NoKeysAddedToObjectErr|Options(?:DataIsOrderedMask|NoneMask|ReleaseStringMask)|SearchListErr)|extBreak(?:C(?:harMask|lusterMask)|GoBackwardsMask|IterateMask|L(?:eadingEdgeMask|ineMask|ocatorMissingType)|ParagraphMask|WordMask)|oken(?:NotFound|izer(?:IterationFinished|UnknownLang))|ypeSelectMaxListSize))|I(?:Mode(?:All(?:Hidden|Suppressed)|Content(?:Hidden|Suppressed)|Normal)|Option(?:A(?:nimateMenuBar|utoShowMenuBar)|Disable(?:AppleMenu|ForceQuit|Hide|MenuBarTransparency|ProcessSwitch|SessionTerminate)))|RL(?:68kNotSupportedError|A(?:bort(?:Initiated(?:Event|Mask)|ingState)|ccessNotAvailableError|ll(?:BufferEventsMask|EventsMask|NonBufferEventsMask)|uthenticationError)|BinHexFileFlag|Co(?:mpleted(?:Event(?:Mask)?|State)|nnectingState)|D(?:ataAvailable(?:Event(?:Mask)?|State)|e(?:binhexOnlyFlag|stinationExistsError)|i(?:rectoryListingFlag|splay(?:AuthFlag|ProgressFlag))|o(?:Not(?:DeleteOnErrorFlag|TryAnonymousFlag)|wnloading(?:Event|Mask|State)))|E(?:rrorOccurred(?:Event(?:Mask)?|State)|x(?:pand(?:AndVerifyFlag|FileFlag)|tensionFailureError))|FileEmptyError|I(?:n(?:itiat(?:edEvent(?:Mask)?|ingState)|valid(?:C(?:allError|onfigurationError)|URL(?:Error|ReferenceError)))|sDirectoryHintFlag)|LookingUpHostState|N(?:oAutoRedirectFlag|ullState)|P(?:er(?:centEvent(?:Mask)?|iodicEvent(?:Mask)?)|ro(?:gressAlreadyDisplayedError|perty(?:BufferTooSmallError|ChangedEvent(?:Mask)?|NotYetKnownError)))|Re(?:placeExistingFlag|s(?:ervedFlag|ourceFound(?:Event(?:Mask)?|State)|umeDownloadFlag))|S(?:erverBusyError|ystemEvent(?:Mask)?)|TransactionComplete(?:Event(?:Mask)?|State)|U(?:n(?:knownPropertyError|s(?:ettablePropertyError|upportedSchemeError))|pload(?:Flag|ing(?:Event|Mask|State))))|SB(?:A(?:bortedError|lreadyOpenErr)|B(?:adDispatchTable|itstufErr|uf(?:OvrRunErr|UnderRunErr))|C(?:RCErr|ompletionError)|D(?:ataToggleErr|evice(?:Busy|Disconnected|Err|NotSuspended|PowerProblem|Suspended))|EndpointStallErr|FlagsError|In(?:correctTypeErr|ternal(?:Err|Reserved(?:1(?:0)?|2|3|4|5|6|7|8|9))|validBuffer)|LinkErr|No(?:BandwidthError|De(?:lay|viceErr)|Err|Tran|t(?:Found|Handled|RespondingErr|Sent(?:1Err|2Err)))|O(?:utOfMemoryErr|verRunErr)|P(?:B(?:LengthError|VersionError)|IDCheckErr|ending|ipe(?:IdleError|StalledError)|ortDisabled)|Queue(?:Aborted|Full)|R(?:es(?:1Err|2Err)|qErr)|T(?:imedOut|ooMany(?:PipesErr|TransactionsErr))|Un(?:derRunErr|known(?:DeviceErr|InterfaceErr|Notification|PipeErr|RequestErr))|WrongPIDErr)|TC(?:DefaultOptions|OverflowErr|UnderflowErr)|YVY422PixelFormat|n(?:connectedSelector|icode(?:16BitFormat|32BitFormat|ByteOrderMark|C(?:anonical(?:CompVariant|DecompVariant)|ollationClass)|D(?:e(?:compositionType|faultDirection(?:Mask)?)|irectionality(?:Bits|Mask)|ocument(?:InterfaceType)?)|F(?:allback(?:Custom(?:First|Only)|Default(?:First|Only)|InterruptSafeMask|Sequencing(?:Bits|Mask))|orceASCIIRange(?:Bit|Mask))|HFSPlus(?:CompVariant|DecompVariant)|Keep(?:Info(?:Bit|Mask)|SameEncoding(?:Bit|Mask))|L(?:eftToRight(?:Mask)?|ooseMappings(?:Bit|Mask))|Ma(?:pLineFeedToReturn(?:Bit|Mask)|tch(?:Other(?:Base(?:Bit|Mask)|Format(?:Bit|Mask)|Variant(?:Bit|Mask))|Unicode(?:Base(?:Bit|Mask)|Format(?:Bit|Mask)|Variant(?:Bit|Mask)))|xDecomposedVariant)|No(?:Co(?:mp(?:atibilityVariant|osedVariant)|rporateVariant)|HalfwidthChars(?:Bit|Mask)|Subset|rmalizationForm(?:C|D)|t(?:AChar|FromInputMethod))|ObjectReplacement|R(?:eplacementChar|ightToLeft(?:Mask)?)|S(?:CSUFormat|tringUnterminated(?:Bit|Mask)|wappedByteOrderMark)|Text(?:BreakClass|Run(?:Bit|Heuristics(?:Bit|Mask)|Mask))|U(?:TF(?:16(?:BEFormat|Format|LEFormat)|32(?:BEFormat|Format|LEFormat)|7Format|8Format)|se(?:ExternalEncodingForm(?:Bit|Mask)|Fallbacks(?:Bit|Mask)|HFSPlusMapping|LatestMapping))|VerticalForm(?:Bit|Mask))|known(?:Exception|FSObjectIcon|Language|Script)|lock(?:KCEvent(?:Mask)?|StateKCStatus|edIcon)|mappedMemoryException|resolvablePageFaultException|supported(?:CardErr|FunctionErr|ModeErr|VsErr)|wrapKCItemAttr)|p(?:ArrowCharCode|date(?:A(?:E(?:TE|UT)|ctiveInputArea)|KCEvent(?:Mask)?)|per(?:AndLowerCaseSelector|Case(?:NumbersSelector|PetiteCapsSelector|SmallCapsSelector|Type)))|se(?:AtoB|B(?:estGuess|to(?:A|B))|CurrentISA|NativeISA|Pr(?:emadeThread|ofileIntent)|WidePositioning|r(?:D(?:ialogItem|omain)|FolderIcon|I(?:DiskIcon|con)|NameAndPasswordFlag|P(?:PDDomain|referredAlert)|SpecificTmpFolderType|sFolder(?:Icon|Type)))|tilit(?:iesFolder(?:Icon|Type)|yWindowClass))|V(?:CBFlags(?:H(?:FSPlusAPIs(?:Bit|Mask)|ardwareGone(?:Bit|Mask))|IdleFlush(?:Bit|Mask)|VolumeDirty(?:Bit|Mask))|K_(?:ANSI_(?:0|1|2|3|4|5|6|7|8|9|A|B(?:ackslash)?|C(?:omma)?|D|E(?:qual)?|F|G(?:rave)?|H|I|J|K(?:eypad(?:0|1|2|3|4|5|6|7|8|9|Clear|D(?:ecimal|ivide)|E(?:nter|quals)|M(?:inus|ultiply)|Plus))?|L(?:eftBracket)?|M(?:inus)?|N|O|P(?:eriod)?|Q(?:uote)?|R(?:ightBracket)?|S(?:emicolon|lash)?|T|U|V|W|X|Y|Z)|C(?:apsLock|o(?:mmand|ntrol))|D(?:elete|ownArrow)|E(?:nd|scape)|F(?:1(?:0|1|2|3|4|5|6|7|8|9)?|2(?:0)?|3|4|5|6|7|8|9|orwardDelete|unction)|H(?:elp|ome)|ISO_Section|JIS_(?:Eisu|K(?:ana|eypadComma)|Underscore|Yen)|LeftArrow|Mute|Option|Page(?:Down|Up)|R(?:eturn|ight(?:Arrow|Co(?:mmand|ntrol)|Option|Shift))|S(?:hift|pace)|Tab|UpArrow|Volume(?:Down|Up))|LibTag2|arispeedParam_Playback(?:Cents|Rate)|er(?:ifyKCItemAttr|tical(?:Constraint|FractionsSelector|PositionType|SubstitutionType|TabCharCode))|o(?:icesFolder(?:Icon|Type)|lume(?:KCItemAttr|RootFolderType|SettingsFolderType)))|W(?:akeToDoze|hereToEmptyTrashFolderType|i(?:d(?:ePosOffsetBit|getsFolderType)|ndow(?:A(?:ctivationScope(?:All|Independent|None)|lertP(?:osition(?:MainScreen|On(?:MainScreen|ParentWindow(?:Screen)?)|ParentWindow(?:Screen)?)|roc)|syncDragAttribute)|BoundsChange(?:OriginChanged|SizeChanged|User(?:Drag|Resize)|Zoom)|C(?:a(?:n(?:BeVisibleWithoutLoginAttribute|Collapse|DrawInCurrentPort|G(?:etWindowRegion|row)|MeasureTitle|SetupProxyDragImage|Zoom)|scade(?:On(?:MainScreen|ParentWindow(?:Screen)?)|StartAtParentWindowScreen))|enter(?:MainScreen|On(?:MainScreen|ParentWindow(?:Screen)?)|ParentWindow(?:Screen)?)|loseBox(?:Attribute|Rgn)|o(?:llapseBox(?:Attribute|Rgn)|mpositingAttribute|n(?:strain(?:AllowPartial|CalcOnly|M(?:ayResize|ove(?:Minimum|RegardlessOfFit))|StandardOptions|Use(?:SpecifiedBounds|TransitionWindow))|tentRgn)))|D(?:ef(?:HIView|ObjectClass|Proc(?:ID|Ptr|Type)|SupportsColorGrafPort|aultPosition|initionVersion(?:One|Two))|ialogDefProcResID|o(?:cument(?:DefProcResID|Proc)|esNotCycleAttribute)|ra(?:gRgn|wer(?:Clos(?:ed|ing)|Open(?:ing)?)))|Edge(?:Bottom|Default|Left|Right|Top)|F(?:adeTransitionEffect|loat(?:FullZoom(?:GrowProc|Proc)|GrowProc|HorizZoom(?:GrowProc|Proc)|Proc|Side(?:FullZoom(?:GrowProc|Proc)|GrowProc|HorizZoom(?:GrowProc|Proc)|Proc|VertZoom(?:GrowProc|Proc))|VertZoom(?:GrowProc|Proc))|rameworkScaledAttribute|ullZoom(?:Attribute|DocumentProc|GrowDocumentProc))|G(?:enieTransitionEffect|lobalPortRgn|ro(?:up(?:Attr(?:FixedLevel|HideOnCollapse|LayerTogether|MoveTogether|PositionFixed|S(?:elect(?:AsLayer|able)|haredActivation)|ZOrderFixed)|Contents(?:Re(?:curse|turnWindows)|Visible)|Level(?:Active|Inactive|Promoted))|w(?:DocumentProc|Rgn)))|H(?:as(?:RoundBottomBarCornersAttribute|TitleBar)|i(?:de(?:On(?:FullScreenAttribute|SuspendAttribute)|TransitionAction)|ghResolutionCapableAttribute)|oriz(?:Zoom(?:DocumentProc|GrowDocumentProc)|ontalZoomAttribute))|I(?:gnoreClicksAttribute|nWindowMenuAttribute|s(?:Alert|CollapsedState|Modal|Opaque))|L(?:atentVisible(?:AppHidden|Collapsed(?:Group|Owner)|F(?:loater|ullScreen)|Suspend)|iveResizeAttribute)|M(?:e(?:nuIncludeRotate|tal(?:Attribute|NoContentSeparatorAttribute))|o(?:dal(?:DialogProc|ity(?:AppModal|None|SystemModal|WindowModal))|v(?:able(?:AlertProc|Modal(?:DialogProc|GrowProc))|eTransitionAction))|sg(?:C(?:alculateShape|leanUp)|Dra(?:gHilite|w(?:Grow(?:Box|Outline)|InCurrentPort)?)|Get(?:Features|GrowImageRegion|Region)|HitTest|Initialize|M(?:easureTitle|odified)|S(?:etupProxyDragImage|tateChanged)))|No(?:A(?:ctivatesAttribute|ttributes)|ConstrainAttribute|Position|ShadowAttribute|TitleBarAttribute|UpdatesAttribute)|O(?:paque(?:ForEventsAttribute|Rgn)|verlayProc)|P(?:aintProcOptionsNone|lainDialogProc|ropertyPersistent)|Resiz(?:ableAttribute|eTransitionAction)|S(?:h(?:adowDialogProc|eet(?:Alert(?:DefProcResID|Proc)|DefProcResID|Proc|TransitionEffect)|owTransitionAction)|i(?:deTitlebarAttribute|mple(?:DefProcResID|FrameProc|Proc))|lideTransitionEffect|t(?:a(?:gger(?:MainScreen|ParentWindow(?:Screen)?)|ndard(?:DocumentAttributes|FloatingAttributes|HandlerAttribute)|teTitleChanged)|ructureRgn)|upports(?:DragHilite|GetGrowImageRegion|ModifiedBit))|T(?:exturedSquareCornersAttribute|itle(?:BarRgn|ProxyIconRgn|TextRgn)|oolbarButton(?:Attribute|Rgn))|U(?:nifiedTitleAndToolbarAttribute|pdateRgn|tility(?:DefProcResID|SideTitleDefProcResID))|Vert(?:Zoom(?:DocumentProc|GrowDocumentProc)|icalZoomAttribute)|WantsDisposeAtProcessDeath|Zoom(?:BoxRgn|TransitionEffect)|sLatin1(?:PalmVariant|StandardVariant)))|or(?:d(?:FinalSwashesO(?:ffSelector|nSelector)|InitialSwashesO(?:ffSelector|nSelector))|kgroupFolderIcon)|r(?:PermKCStatus|apKCItemAttr|ite(?:FailureErr|ProtectedErr|Reference)))|X(?:86(?:ISA|RTA)|Lib(?:Tag1|Version))|Y(?:UV(?:211PixelFormat|411PixelFormat|SPixelFormat|UPixelFormat)|V(?:U9PixelFormat|YU422PixelFormat))|Zoom(?:Accelerate|Decelerate|NoAcceleration)|administratorUser|e(?:rnel(?:A(?:lreadyFreeErr|sync(?:ReceiveLimitErr|SendLimitErr)|ttributeErr)|CanceledErr|DeletePermissionErr|Ex(?:ceptionErr|ecut(?:ePermissionErr|ionLevelErr))|I(?:DErr|n(?:UseErr|completeErr))|O(?:bjectExistsErr|ptionsErr)|PrivilegeErr|Re(?:adPermissionErr|turnValueErr)|T(?:erminatedErr|imeoutErr)|Un(?:recoverableErr|supportedErr)|WritePermissionErr)|y(?:32BitIcon|4BitIcon|8Bit(?:Icon|Mask)|A(?:E(?:A(?:djustMarksProc|ngle|rcAngle|ttaching)|B(?:aseAddr|estType|gnd(?:Color|Pattern)|ounds|ufferSize)|C(?:ellList|la(?:ssID|useOffsets)|o(?:lor(?:Table)?|mp(?:Operator|areProc)|ntainer|untProc)|ur(?:rentPoint|ve(?:Height|Width)))|D(?:a(?:shStyle|ta)|e(?:f(?:aultType|initionRect)|s(?:cType|iredClass|tination))|o(?:AntiAlias|Dithered|Rotate|Scale|Translate)|ragging)|E(?:ditionFileLoc|lements|ndPoint|rrorObject|vent(?:Class|ID))|F(?:i(?:l(?:e(?:Type)?|l(?:Color|Pattern))|xLength)|lip(?:Horizontal|Vertical)|o(?:nt|rmula))|G(?:etErrDescProc|raphicObjects)|H(?:iliteRange|omograph(?:Accent|DicInfo|Weight))|I(?:D|mageQuality|n(?:dex|sertHere))|Key(?:Data|Form(?:s)?|word)|L(?:A(?:Homograph|Morpheme(?:Bundle|Path)?)|aunchedAs(?:LogInItem|ServiceItem)|e(?:ftSide|vel)|ineArrow|ogical(?:Operator|Terms))|M(?:ark(?:Proc|TokenProc)|o(?:rpheme(?:PartOfSpeechCode|TextRange)|veView))|N(?:ame|e(?:wElementLoc|xtBody)|oAutoRouting)|O(?:bject(?:1|2|Class)?|ff(?:Styles|set)|nStyles)|P(?:MTable|OSTHeaderData|aram(?:Flags|eters)|en(?:Color|Pattern|Width)|i(?:nRange|x(?:MapMinus|elDepth))|o(?:int(?:List|Size)?|sition)|ro(?:p(?:Data|Flags|ID|ert(?:ies|y))|tection))|R(?:angeSt(?:art|op)|e(?:corderCount|gionClass|nderAs|pl(?:acing|yHeaderData)|questedType|sult(?:Info)?)|o(?:t(?:Point|ation)|wList))|S(?:aveOptions|c(?:ale|riptTag)|e(?:archText|rverInstance)|howWhere|t(?:art(?:Angle|Point)|yles)|uiteID)|T(?:SM(?:DocumentRefcon|EventRe(?:cord|f)|GlyphInfoArray|ScriptTag|Text(?:F(?:MFont|ont)|PointSize))|arget|e(?:st|xt(?:Color|Font|Line(?:Ascent|Height)|PointSize|S(?:ervice(?:Encoding|MacEncoding)|tyles))?)|he(?:Data|Text)|r(?:ans(?:ferMode|lation)|yAsStructGraf))|U(?:niformStyles|pdate(?:On|Range)|s(?:erTerm|ing))|Version|W(?:hoseRangeSt(?:art|op)|indow|ritingCode)|XMLRe(?:plyData|questData))|S(?:Arg|P(?:ositionalArgs|reposition(?:A(?:bo(?:ut|ve)|gainst|partFrom|round|sideFrom|t)|B(?:e(?:low|neath|side|tween)|y)|F(?:or|rom)|Given|Has|In(?:steadOf|to)?|O(?:n(?:to)?|utOf|ver)|Since|T(?:hr(?:ough|u)|o)|Un(?:der|til)|With(?:out)?))|Returning|SubroutineName|UserRecordFields)|c(?:ceptTimeoutAttr|tualSenderAuditToken)|dd(?:itionalHTTPHeaders|ressAttr)|ll|pp(?:HandledCoercion|leEventAttributesAttr))|C(?:loseAllWindows|o(?:deMask|ntextualMenu(?:Attributes|CommandID|Modifiers|Name|Submenu)))|D(?:CM(?:Field(?:Attributes|DefaultData|FindMethods|Name|T(?:ag|ype))|MaxRecordSize)|i(?:rectObject|s(?:ableAuthenticationAttr|poseTokenProc))|own(?:Mask)?|riveNumber)|E(?:rror(?:Code|Number|String)|v(?:ent(?:ClassAttr|IDAttr|SourceAttr)|tDev))|GlobalPositionList|HighLevel(?:Class|ID)|I(?:CEditPreferenceDestination|conAndMask|nteractLevelAttr)|Key(?:Code|board)?|Local(?:PositionList|Where)|M(?:enuI(?:D|tem)|i(?:ni(?:1BitMask|4BitIcon|8BitIcon)|s(?:cellaneous|sedKeywordAttr))|odifiers)|NewBounds|O(?:SA(?:Dialect(?:Code|LangCode|Name|ScriptCode)|Source(?:End|Start))|ldFinderItems|ptionalKeywordAttr|riginal(?:AddressAttr|Bounds))|Pr(?:eDispatch|ocessSerialNumber)|R(?:PCMethod(?:Name|Param(?:Order)?)|e(?:directedDocumentList|ply(?:PortAttr|RequestedAttr)|turnIDAttr))|S(?:OAP(?:Action|MethodNameSpace(?:URI)?|S(?:MD(?:Namespace(?:URI)?|Type)|chemaVersion|tructureMetaData))|R(?:Recognizer|Speech(?:Result|Status))|cszResource|e(?:lect(?:Proc|ion)|nder(?:A(?:ppl(?:escriptEntitlementsAttr|ication(?:IdentifierEntitlementAttr|Sandboxed))|uditTokenAttr)|E(?:GIDAttr|UIDAttr)|GIDAttr|PIDAttr|UIDAttr))|mall(?:32BitIcon|4BitIcon|8Bit(?:Icon|Mask)|IconAndMask)|ubjectAttr)|T(?:imeoutAttr|ransactionIDAttr)|U(?:p(?:Mask)?|ser(?:NameAttr|PasswordAttr))|W(?:he(?:n|re)|indow)|XMLDebuggingAttr))|fullPrivileges|i(?:Movie(?:FolderType|PlugInsFolderType|SoundEffectsFolderType)|o(?:AC(?:Access(?:BlankAccess(?:Bit|Mask)|Everyone(?:Read(?:Bit|Mask)|Search(?:Bit|Mask)|Write(?:Bit|Mask))|Group(?:Read(?:Bit|Mask)|Search(?:Bit|Mask)|Write(?:Bit|Mask))|Owner(?:Bit|Mask|Read(?:Bit|Mask)|Search(?:Bit|Mask)|Write(?:Bit|Mask))|User(?:Read(?:Bit|Mask)|Search(?:Bit|Mask)|Write(?:Bit|Mask)))|UserNo(?:MakeChanges(?:Bit|Mask)|SeeF(?:iles(?:Bit|Mask)|older(?:Bit|Mask))|tOwner(?:Bit|Mask)))|F(?:CB(?:FileLocked(?:Bit|Mask)|LargeFile(?:Bit|Mask)|Modified(?:Bit|Mask)|OwnClump(?:Bit|Mask)|Resource(?:Bit|Mask)|SharedWrite(?:Bit|Mask)|Write(?:Bit|Locked(?:Bit|Mask)|Mask))|lAttrib(?:CopyProt(?:Bit|Mask)|D(?:ataOpen(?:Bit|Mask)|ir(?:Bit|Mask))|FileOpen(?:Bit|Mask)|InShared(?:Bit|Mask)|Locked(?:Bit|Mask)|Mounted(?:Bit|Mask)|ResOpen(?:Bit|Mask)|SharePoint(?:Bit|Mask)))|VAtrb(?:DefaultVolume(?:Bit|Mask)|FilesOpen(?:Bit|Mask)|HardwareLocked(?:Bit|Mask)|SoftwareLocked(?:Bit|Mask))))|no(?:Group|User)|ownerPrivileges)|l(?:CloseMsg|D(?:o(?:HAutoscroll(?:Bit)?|VAutoscroll(?:Bit)?)|raw(?:Msg|ingModeOff(?:Bit)?))|ExtendDrag(?:Bit)?|HiliteMsg|InitMsg|No(?:Disjoint(?:Bit)?|Extend(?:Bit)?|NilHilite(?:Bit)?|Rect(?:Bit)?)|OnlyOne(?:Bit)?|UseSense(?:Bit)?|a(?:Dictionary(?:NotOpenedErr|TooManyErr|UnknownErr)|En(?:gineNotFoundErr|vironment(?:BusyErr|ExistErr|NotFoundErr))|FailAnalysisErr|InvalidPathErr|NoMoreMorphemeErr|Property(?:Err|IsReadOnlyErr|NotFoundErr|UnknownErr|ValueErr)|T(?:extOverFlowErr|ooSmallBufferErr)|ng(?:A(?:fri(?:caans|kaans)|lbanian|mharic|r(?:abic|menian)|ssamese|ymara|zerbaijan(?:Ar|Roman|i))|B(?:asque|e(?:lorussian|ngali)|reton|u(?:lgarian|rmese)|yelorussian)|C(?:atalan|h(?:ewa|inese)|roatian|zech)|D(?:anish|utch|zongkha)|E(?:nglish|s(?:peranto|tonian))|F(?:a(?:eroese|r(?:oese|si))|innish|lemish|rench)|G(?:al(?:ician|la)|e(?:orgian|rman)|ree(?:k(?:Ancient|Poly)?|nlandic)|u(?:arani|jarati))|H(?:ebrew|indi|ungarian)|I(?:celandic|n(?:donesian|uktitut)|rish(?:Gaelic(?:Script)?)?|talian)|Ja(?:panese|vaneseRom)|K(?:a(?:nnada|shmiri|zakh)|hmer|i(?:nyarwanda|rghiz)|orean|urdish)|L(?:a(?:o|pp(?:ish|onian)|t(?:in|vian))|ettish|ithuanian)|M(?:a(?:cedonian|l(?:a(?:gasy|y(?:Arabic|Roman|alam))|t(?:a|ese))|nxGaelic|rathi)|o(?:ldavian|ngolian(?:Cyr)?))|N(?:epali|orwegian|y(?:anja|norsk))|Or(?:iya|omo)|P(?:ashto|ersian|o(?:lish|rtug(?:ese|uese))|unjabi)|Quechua|R(?:omanian|u(?:anda|ndi|ssian))|S(?:a(?:amisk|mi|nskrit)|cottishGaelic|erbian|i(?:mpChinese|n(?:dhi|halese))|lov(?:ak|enian)|omali|panish|undaneseRom|w(?:ahili|edish))|T(?:a(?:galog|jiki|mil|tar)|elugu|hai|i(?:betan|grinya)|ongan|radChinese|urk(?:ish|men))|U(?:ighur|krainian|nspecified|rdu|zbek)|Vietnamese|Welsh|Y(?:iddish|ugoslavian))|pProtErr|rge(?:1BitMask|4BitData|8BitData)|stDskErr|unch(?:Allow24Bit|Continue|DontSwitch|InhibitDaemon|NoFileFlags|UseMinimum))|eft(?:OverChars|SingGuillemet)|imitReachedErr|o(?:c(?:alOnlyErr|kPortBits(?:Bad(?:PortErr|SurfaceErr)|SurfaceLostErr|W(?:indow(?:ClippedErr|MovedErr|ResizedErr)|rongGDeviceErr)))|ng(?:Da(?:te(?:Found)?|y)|Month|Week|Year)))|m(?:BarNFnd|CalcItemMsg|D(?:ownMask|rawMsg)|FulErr|PopUpMsg|SizeMsg|UpMask|a(?:c(?:Dev|ron)|p(?:C(?:hanged(?:Bit)?|ompact(?:Bit)?)|Read(?:Err|Only(?:Bit)?))|trixErr|x(?:Country|DateField|SizeToGrowTooSmall))|ct(?:AllItems|LastIDIndic)|dy|e(?:diaTypesDontMatch|m(?:A(?:ZErr|drErr)|BCErr|F(?:ragErr|ullErr)|LockedErr|P(?:CErr|urErr)|ROZ(?:Err(?:or)?|Warn)|SCErr|WZErr)|nu(?:I(?:nvalidErr|temNotFoundErr)|NotFoundErr|Pr(?:gErr|operty(?:Invalid(?:Err)?|NotFoundErr))|UsesSystemDefErr))|i(?:di(?:DupIDErr|InvalidCmdErr|ManagerAbsentOSErr|N(?:ameLenErr|o(?:C(?:lientErr|onErr)|PortErr))|TooMany(?:ConsErr|PortsErr)|VConnect(?:Err|Made|Rmvd)|WriteErr)|n(?:Country|LeadingZ|i(?:1BitMask|4BitData|8BitData)|ute(?:Field|Mask))|ssingRequiredParameterErr)|mInternalError|ntLdingZ|o(?:de(?:32BitCompatible|C(?:anBackground|ontrolPanel)|D(?:eskAccessory|isplayManagerAware|oesActivateOnFGSwitch)|Get(?:AppDiedMsg|FrontClicks)|HighLevelEventAware|L(?:aunchDontSwitch|iteral|ocalAndRemoteHLEvents)|MultiLaunch|N(?:eedSuspendResume|ormal)|OnlyBackground|Phonemes|Reserved|StationeryAware|T(?:ext|une)|UseTextEditServices)|nth(?:Field|Mask)|u(?:ntedFolderIconResource|se(?:Down|MovedMessage|Up))|v(?:ableDBoxProc|ieT(?:extNotFoundErr|oolboxUninitialized)))|pWorkFlag(?:CopyWorkBlock|Do(?:Completion|Work|ntBlock)|Get(?:IsRunning|ProcessorCount))|ultiplePublisherWrn|yd)|n(?:WIDTHHook|ame(?:FontTableTag|TypeErr)|bp(?:BuffOvr|ConfDiff|Duplicate|N(?:ISErr|o(?:Confirm|tFound)))|e(?:edClearScrapErr|gZcbFreeErr|twork(?:E(?:rr|vt)|Mask)|wLine(?:Bit|CharMask|Mask))|il(?:HandleErr|ScrapFlavorDataErr)|mTyp(?:Err|e)|o(?:AdrMkErr|BridgeErr|C(?:a(?:che(?:Bit|Mask)|lls)|o(?:decErr|nstraint))|D(?:MAErr|ata(?:Area|Handler)|e(?:fault(?:DataRef|UserErr)|viceForChannel)|riveErr|taMkErr)|ExportProcAvailableErr|G(?:lobalsErr|rowDocProc)|H(?:ardware(?:Err)?|elpForItem)|I(?:conDataAvailableErr|nformErr)|M(?:MUErr|PPErr|a(?:c(?:DskErr|hineNameErr)|rk|skFoundErr)|e(?:diaHandler|m(?:ForPictPlaybackErr|oryNodeFailedInitialize))|o(?:re(?:FolderDescErr|KeyColorsErr|RealTime)|vieFound))|NybErr|OutstandingHLE|P(?:a(?:steboardPromiseKeeperErr|thMappingErr)|ortErr|refAppErr)|Re(?:cordOfApp|lErr|quest|sponseErr)|S(?:crap(?:Err|PromiseKeeperErr)|e(?:curitySession|ndResp|ssionErr)|ou(?:ndTrackInMovieErr|rceTreeFoundErr)|u(?:chIconErr|itableDisplaysErr)|ynthFound)|T(?:humbnailFoundErr|oolboxNameErr|ranslationPathErr|ypeErr)|User(?:InteractionAllowed|NameErr|Re(?:cErr|fErr))|VideoTrackInMovieErr|n(?:DragOriginatorErr|GlyphID|MatchingEditState)|t(?:A(?:FileErr|QTVRMovieErr|RemountErr|llowedToSaveMovieErr|ppropriateForClassic)|BTree|E(?:nough(?:BufferSpace|D(?:ataErr|iskSpaceToGrab)|Hardware(?:Err)?|Memory(?:Err|ToGrab))|xact(?:MatrixErr|SizeErr))|HeldErr|I(?:mplementedMusicOSErr|nitErr)|L(?:eafAtomErr|o(?:ckedErr|ggedInErr))|OpenErr|PasteboardOwnerErr|RegisteredSectionErr|ThePublisherWrn|e(?:ChannelNotAllocatedOSErr|Icon)))|r(?:CallNotSupported|DataTruncatedErr|ExitedIteratorScope|I(?:nvalid(?:EntryIterationOp|NodeErr)|terationDone)|LockedErr|N(?:ameErr|ot(?:CreatedErr|EnoughMemoryErr|FoundErr|ModifiedErr|SlotDeviceErr))|OverrunErr|P(?:ath(?:BufferTooSmall|NotFound)|ower(?:Err|SwitchAbortErr)|ropertyAlreadyExists)|ResultCodeBase|T(?:ransactionAborted|ypeMismatchErr))|s(?:DrvErr|StackErr|vErr)|u(?:l(?:Dev|lEvent)|mberFor(?:matting(?:Bad(?:CurrencyPositionErr|FormatErr|NumberFormattingObjectErr|OptionsErr|TokenErr)|DelimiterMissingErr|EmptyFormatErr|LiteralMissingErr|NotA(?:DigitErr|NumberErr)|OverflowInDestinationErr|SpuriousCharErr|UnOrd(?:eredCurrencyRangeErr|redCurrencyRangeErr))|tmattingNotADigitErr)))|o(?:ffLinErr|gonek|k|p(?:WrErr|en(?:Err|FolderIconResource)|tionKey(?:Bit)?)|s(?:2FontTableTag|Evt(?:MessageMask)?|Mask)|ver(?:Dot|layDITL)|wnedFolderIconResource)|p(?:A(?:RADialIn|S(?:Da(?:teString|y(?:s)?)|Hours|It|M(?:e|inutes|onth)|P(?:arent|i|rint(?:Depth|Length))|Quote|Re(?:quiredImportItems|sult|turn)|S(?:econds|pace)|T(?:ab|ime(?:String)?|opLevelScript)|Week(?:day|s)|Year)|T(?:Machine|Type|Zone)|boutMacintosh|pp(?:Partition|l(?:eMenuItemsFolder|icationFile))|r(?:cAngle|ePrivilegesInherited))|B(?:ackground(?:Color|Pattern)|estType|ounds|uttonViewArrangement|y(?:CreationDateArrangement|KindArrangement|LabelArrangement|ModificationDateArrangement|NameArrangement|SizeArrangement))|C(?:a(?:llBackNumber|n(?:C(?:hangePassword|onnect)|DoProgramLinking)|pacity)|l(?:ass|ipboard)|o(?:lor(?:Table)?|m(?:ment|pletelyExpanded)|n(?:duit|t(?:ainer(?:Window)?|ent(?:Space|s)|rolPanelsFolder))|rnerCurve(?:Height|Width))|reationDate(?:Old)?)|D(?:CM(?:AccessMethod|C(?:lass|opyright)|L(?:isting|ocale)|Maintenance|Permission)|NS(?:Form)?|ashStyle|e(?:f(?:ault(?:ButtonViewIconSize|IconViewIconSize|ListViewIconSize|Type)|initionRect)|layBeforeSpringing|s(?:cription|k(?:AccessoryFile|top))|vice(?:Address|Type))|isk|ottedDecimal)|E(?:jectable|n(?:abled|dPoint|tireContents)|x(?:p(?:and(?:able|ed)|orted)|tensionsFolder))|F(?:TPKind|i(?:l(?:e(?:Creator|Share(?:On|StartingUp)|Type(?:Old)?)?|l(?:Color|Pattern))|nderPreferences)|o(?:lder(?:Old)?|nt(?:sFolder(?:PreAllegro)?)?|rmula)|reeSpace)|G(?:r(?:aphicObjects|id(?:Icons)?|oup(?:Privileges)?)|uestPrivileges)|H(?:as(?:CloseBox|ScriptingTerminology|TitleBar)|ost)|I(?:D|con(?:Bitmap|Size|ViewArrangement)|n(?:dex|fo(?:Panel|Window)|herits|sertionLoc|ternetLocation)|s(?:Collapsed|F(?:loating|rontProcess)|Locked(?:Old)?|Mod(?:al|ified)|Owner|P(?:opup|ulledOpen)|Resizable|S(?:criptable|elected|ta(?:rtup|tioneryPad))|Zoom(?:able|ed(?:Full)?))|temNumber)|Justification|K(?:e(?:epArranged(?:By)?|y(?:Kind|strokeKey))|ind)|L(?:a(?:bel(?:1|2|3|4|5|6|7|Index)|ngCode|rge(?:Button|stFreeBlock))|ength|i(?:neArrow|stViewIconSize)|ocal(?:AndRemoteEvents)?)|M(?:akeChanges|enuID|inAppPartition|o(?:difi(?:cationDate(?:Old)?|ers)|unted))|N(?:ame|e(?:twork|wElementLoc)|o(?:Arrangement|de))|O(?:bject|riginalItem|wner(?:Privileges)?)|P(?:a(?:rtitionSpaceUsed|th)|en(?:Color|Pattern|Width)|hysicalSize|ixelDepth|o(?:int(?:List|Size)|rt|sition)|r(?:e(?:ferences(?:Folder|Window)|viousView)|o(?:ductVersion|gramLinkingOn|perties|t(?:ection|ocol))))|R(?:e(?:st|verse)|otation)|S(?:CSI(?:Bus|LUN)|c(?:ale|heme|ript(?:Code|Tag)?)|e(?:eF(?:iles|olders)|lect(?:ed|ion))|h(?:ar(?:ableContainer|ing(?:Protection|Window)?)|o(?:rtCuts|uldCallBack|w(?:C(?:omment|reationDate)|D(?:ate|iskInfo)|FolderSize|Kind|Label|ModificationDate|Size|Version))|utdownFolder)|ize|mall(?:Button|Icon)|napToGridArrangement|o(?:cket|rtDirection|und)|pringOpenFolders|ta(?:ggerIcons|rt(?:Angle|Point|ingUp|up(?:Disk|ItemsFolder)))|uggestedAppPartition|ystemFolder)|T(?:e(?:mporaryFolder|xt(?:Color|Encoding|Font|ItemDelimiters|PointSize|Styles))|ra(?:ns(?:ferMode|lation)|sh))|U(?:RL|niformStyles|pdateOn|se(?:RelativeDate|ShortMenus|WideGrid|r(?:Name|Password|Selection)))|V(?:ersion|i(?:ew(?:Font(?:Size)?|Preferences)?|sible))|W(?:arnOnEmpty|indow)|a(?:ramErr|steDev|th(?:NotVerifiedErr|TooLongErr))|er(?:Thousand|mErr)|i(?:c(?:Item|ker(?:CantLive|ResourceError)|t(?:Info(?:IDErr|Ver(?:bErr|sionErr))|ureDataErr))|xMapTooDeepErr)|l(?:a(?:inDBox|tform(?:68k|AIXppc|I(?:A32NativeEntryPoint|RIXmips|nterpreted)|Linux(?:intel|ppc)|MacOSx86|NeXT(?:68k|Intel|ppc|sparc)|PowerPC(?:64NativeEntryPoint|NativeEntryPoint)?|SunOS(?:intel|sparc)|Win32|X86_64NativeEntryPoint))|easeCache(?:Bit|Mask))|m(?:BusyErr|Field|Mask|Re(?:cv(?:EndErr|StartErr)|plyTOErr)|Send(?:EndErr|StartErr))|o(?:pup(?:FixedWidth|MenuProc|Title(?:Bold|C(?:enterJust|ondense)|Extend|Italic|LeftJust|NoStyle|Outline|RightJust|Shadow|Underline)|Use(?:AddResMenu|WFont)|VariableWidth)|rt(?:ClosedErr|InUse|N(?:ameExistsErr|ot(?:Cf|Pwr)))|sErr)|r(?:InitErr|WrErr|eferencesFolderIconResource|i(?:nt(?:MonitorFolderIconResource|erStatusOpCodeNotSupportedErr)|vateFolderIconResource)|o(?:c(?:NotFound|essStateIncorrectErr)|gressProcAborted|pertyNotSupportedByNodeErr|tocolErr))|ushButProc)|q(?:Err|fcbNot(?:CreatedErr|FoundErr)|t(?:ActionNotHandledErr|NetworkAlreadyAllocatedErr|ParamErr|XML(?:ApplicationErr|ParseErr)|ml(?:Dll(?:EntryNotFoundErr|LoadErr)|Uninitialized)|s(?:AddressBusyErr|Bad(?:DataErr|S(?:electorErr|tateErr))|ConnectionFailedErr|T(?:imeoutErr|ooMuchDataErr)|Un(?:knownValueErr|supported(?:DataTypeErr|FeatureErr|RateErr)))|vr(?:LibraryLoadErr|Uninitialized))|ueueFull)|r(?:AliasType|ad(?:Ctrl|ioButProc)|c(?:DB(?:AsyncNotSupp|B(?:ad(?:AsyncPB|DDEV|Sess(?:ID|Num)|Type)|reak)|E(?:rror|xec)|N(?:oHandler|ull)|PackNotInited|Value|WrongVersion)|vrErr)|dVerify(?:Bit|Mask)?|e(?:ad(?:Err|QErr|Reference)|c(?:NotFnd|ordDataTooBigErr)|gisterComponent(?:A(?:fterExisting|liasesOnly)|Global|NoDuplicates)|q(?:Aborted|Failed|uiredFlagsDontMatch)|s(?:1Field|2Field|3Field|AttrErr|C(?:hanged(?:Bit)?|trl)|FNotFound|Locked(?:Bit)?|NotFound|P(?:r(?:eload(?:Bit)?|o(?:blem|tected(?:Bit)?))|urgeable(?:Bit)?)|Sys(?:Heap(?:Bit)?|RefBit)|ourceInMemory|umeFlag)|tryComponentRegistrationErr)|fNumErr|gn(?:OverflowErr|TooBigErr(?:or)?)|i(?:ght(?:ControlKey(?:Bit)?|OptionKey(?:Bit)?|S(?:hiftKey(?:Bit)?|ingGuillemet))|ngMark)|mvRe(?:fFailed|sFailed)|o(?:man(?:AppFond|Flags|SysFond)|utingNotFoundErr))|s(?:IQType|am(?:eFileErr|plesAlreadyInMediaErr)|c(?:TypeNotFoundErr|r(?:ap(?:Flavor(?:FlagsMismatchErr|NotFoundErr|SizeMismatchErr)|PromiseNotKeptErr)|ipt(?:CurLang|DefLang)|ollBarProc))|dm(?:InitErr|JTInitErr|P(?:RAMInitErr|riInitErr)|SRTInitErr)|e(?:NoDB|c(?:LeadingZ|ond(?:Field|Mask)|tNFErr)|ekErr|lectorNotSupportedByNodeErr|pNot(?:Consistent|IntlSep)|qGrabInfoNotAvailable|ss(?:ClosedErr|TableErr|ion(?:Has(?:GraphicAccess|TTY)|IsR(?:emote|oot)|KeepCurrentBootstrap))|ttingNotSupportedByNodeErr)|h(?:aredFolderIconResource|iftKey(?:Bit)?|ortDate|utDownAlert)|i(?:Bad(?:DeviceName|RefNum|SoundInDevice)|DeviceBusyErr|HardDriveTooSlow|In(?:it(?:S(?:DTblErr|PTblErr)|VBLQsErr)|putDeviceErr|valid(?:Compression|Sample(?:Rate|Size)))|No(?:BufferSpecified|SoundInHardware)|Unknown(?:InfoType|Quality)|VBRCompressionNotSupported|ze(?:Bit|of_sfnt(?:CMap(?:E(?:ncoding|xtendedSubHeader)|Header|SubHeader)|D(?:escriptorHeader|irectory)|Instance|Name(?:Header|Record)|Variation(?:Axis|Header))))|ktClosedErr|l(?:eepQType|otNumErr|pQType)|m(?:A(?:llScripts|mharic|r(?:abic|menian))|B(?:LFieldBad|ad(?:BoardId|RefId|Script|Verb|s(?:List|PtrErr))|engali|lkMoveErr|u(?:rmese|sErrTO)|yteLanesErr)|C(?:PUErr|RCFail|entralEuroRoman|h(?:ar(?:1byte|2byte|Ascii|B(?:idirect|opomofo)|ContextualLR|E(?:uro|xtAscii)|FIS(?:G(?:ana|reek)|Ideo|Kana|Russian)|GanaKana|H(?:angul|iragana|orizontal)|Ideographic|Jamo|Katakana|L(?:eft|ower)|NonContextualLR|Punct|Right|TwoByte(?:Greek|Russian)|Upper|Vertical)|inese)|kStatusErr|odeRevErr|urrentScript|yrillic)|D(?:evanagari|is(?:DrvrNamErr|abledSlot|posePErr))|E(?:astEurRoman|mptySlot|thiopic|xtArabic)|F(?:HBl(?:kDispErr|ockRdErr)|ISClass(?:Lvl(?:1|2)|User)|irstByte|o(?:nd(?:End|Start)|rmatErr))|G(?:e(?:ez|orgian|t(?:DrvrNamErr|PRErr))|reek|u(?:jarati|rmukhi))|Hebrew|I(?:deographic(?:Level(?:1|2)|User)|nit(?:StatVErr|TblVErr))|Ja(?:mo(?:Bog(?:Jaeum|Moeum)|Jaeum|Moeum)|panese)|K(?:CHRCache|an(?:a(?:HardOK|S(?:mall|oftOK))|nada)|ey(?:DisableKybd(?:Switch|s)|EnableKybds|ForceKeyScript(?:Bit|Mask)|Next(?:InputMethod|Kybd|Script)|Roman|S(?:cript|etDir(?:LeftRight|RightLeft)|wap(?:InputMethod|Kybd|Script)|ysScript)|Toggle(?:Direction|Inline))|hmer|lingon|orean)|La(?:o(?:tian)?|stByte)|M(?:a(?:layalam|sk(?:A(?:ll|scii(?:1|2)?)|Bopomofo2|Gana2|Hangul2|Jamo2|Kana(?:1|2)|Native))|iddleByte|ongolian)|N(?:ewPErr|ilsBlockErr|o(?:Board(?:Id|SRsrc)|Dir|GoodOpens|JmpTbl|MoresRsrcs|sInfoArray|tInstalled)|umberPartsTable)|O(?:ffsetErr|riya)|P(?:RAMInitErr|riInitErr|unct(?:Blank|Graphic|N(?:ormal|umber)|Repeat|Symbol))|R(?:Symbol|e(?:cNotFnd|gionCode|s(?:erved(?:Err|Slot)|rvErr)|visionErr)|oman|ussian)|S(?:DMInitErr|RT(?:InitErr|OvrFlErr)|elOOBErr|i(?:mpChinese|n(?:dhi|gleByte|halese))|l(?:avic|otOOBErr)|ys(?:Script|temScript))|T(?:amil|elugu|hai|ibetan|ra(?:dChinese|ns(?:Ascii(?:1|2)?|Bopomofo2|Case|Gana2|Hangul(?:2|Format)|Jamo2|Kana(?:1|2)|Lower|Native|Pre(?:DoubleByting|LowerCasing)|RuleBaseFormat|System|Upper)))|U(?:n(?:ExBusErr|TokenTable|i(?:codeScript|nterp))|prHalfCharSet)|Vietnamese|W(?:hiteSpaceList|ord(?:SelectTable|WrapTable))|all(?:1BitMask|4BitData|8BitData|DateBit)|c(?:ClassMask|DoubleMask|OrientationMask|R(?:eserved|ightMask)|TypeMask|UpperMask)|f(?:D(?:isableKeyScriptSync(?:Mask)?|ualCaret)|NameTagEnab|ShowIcon|UseAssocFontInfo)|s(?:GetDrvrErr|PointerNil|f(?:AutoInit|B0Digits|Context|Forms|IntellCP|Ligatures|N(?:atCase|oForceFont)|Reverse|S(?:ingByte|ynchUnstyledTE)|UnivExt)))|o(?:C(?:haracterMode|ommandDelimiter|urrent(?:A5|Voice))|Error(?:CallBack|s)|InputMode|NumberMode|OutputTo(?:AudioDevice|ExtAudioFile|FileWithCFURL)|P(?:honeme(?:CallBack|Options|Symbols)|itch(?:Base|Mod))|R(?:ate|e(?:centSync|fCon|set))|S(?:oundOutput|peechDoneCallBack|tatus|yn(?:cCallBack|th(?:Extension|Type)))|TextDoneCallBack|Vo(?:ice(?:Description|File)|lume)|WordCallBack|rts(?:After|Before|Equal)|u(?:ndSupportNotAvailableErr|rceNotFoundErr))|pdAdjErr|rcCopy|t(?:a(?:leEditState|rtupFolderIconResource|t(?:Text|usErr))|opIcon|r(?:UserBreak|eamingNodeNotReadyErr|ingOverflow))|u(?:p(?:Day|Month|Week|Year)|spendResumeMessage)|v(?:All(?:1BitData|4BitData|8BitData|AvailableData|LargeData|MiniData|SmallData)|Disabled|Large(?:1Bit|4Bit|8Bit)|Mini(?:1Bit|4Bit|8Bit)|Small(?:1Bit|4Bit|8Bit)|TempDisable)|y(?:nth(?:NotReady|OpenFailed|esizer(?:NotRespondingOSErr|OSErr))|stem(?:CurLang|DefLang|FolderIconResource)))|t(?:aDst(?:DocNeedsResourceFork|IsAppTranslation)|e(?:Bit(?:Clear|Set|Test)|C(?:aret|enter)|Draw|F(?:AutoScroll|I(?:dleWithEventLoopTimer|nlineInput(?:AutoScroll)?)|OutlineHilite|TextBuffering|Use(?:InlineInput|TextServices|WhiteBackground)|ind|lush(?:Default|Left|Right)|orceLeft|rom(?:Find|Recal))|Highlight|Just(?:Center|Left|Right)|ScrapSizeErr|Word(?:Drag|Select)|l(?:A(?:PattNotSupp|lreadyOpen|utoAnsNotOn)|Bad(?:APattErr|BearerType|C(?:AErr|odeResource)|D(?:N(?:DType|Err|Type)|isplayMode)|F(?:eatureID|unction|wdType)|H(?:TypeErr|andErr)|In(?:dex|t(?:Ext|ercomID))|LevelErr|P(?:a(?:geID|rkID)|ickupGroupID|roc(?:Err|ID))|Rate|S(?:WErr|ampleRate|elect|tateErr)|TermErr|VTypeErr)|C(?:A(?:Not(?:Acceptable|Deflectable|Rejectable)|Unavail)|BErr|onf(?:Err|LimitE(?:rr|xceeded)|NoLimit|Rej))|D(?:N(?:DTypeNotSupp|TypeNotSupp)|e(?:tAlreadyOn|viceNotFound)|isplayModeNotSupp)|F(?:eat(?:Active|Not(?:Avail|Su(?:b|pp)))|wdTypeNotSupp)|GenericError|HTypeNotSupp|In(?:dexNotSupp|itFailed|tExtNotSupp)|No(?:C(?:allbackRef|ommFolder)|Err|MemErr|OpenErr|SuchTool|Tools|tEnoughdspBW)|PBErr|St(?:ateNotSupp|illNeeded)|T(?:ermNotOpen|ransfer(?:Err|Rej))|UnknownErr|V(?:TypeNotSupp|alidateFailed))|xt(?:MenuProc|Parser(?:Bad(?:Par(?:amErr|serObjectErr)|T(?:ext(?:EncodingErr|LanguageErr)|okenValueErr))|No(?:MoreT(?:extErr|okensErr)|SuchTokenFoundErr)|ObjectNotFoundErr|ParamErr)))|h(?:eme(?:Bad(?:CursorIndexErr|TextColorErr)|HasNoAccentsErr|InvalidBrushErr|MonitorDepthNotSupportedErr|NoAppropriateBrushErr|Process(?:NotRegisteredErr|RegisteredErr)|ScriptFontNotFoundErr)|read(?:BadAppContextErr|NotFoundErr|ProtocolErr|TooManyReqsErr))|i(?:lde|me(?:Cycle(?:12|24|Zero)|NotIn(?:Media|Track|ViewErr)))|k0BadErr|ls_(?:ciphersuite_(?:AES_(?:128_GCM_SHA256|256_GCM_SHA384)|CHACHA20_POLY1305_SHA256|ECDHE_(?:ECDSA_WITH_(?:3DES_EDE_CBC_SHA|AES_(?:128_(?:CBC_SHA(?:256)?|GCM_SHA256)|256_(?:CBC_SHA(?:384)?|GCM_SHA384))|CHACHA20_POLY1305_SHA256)|RSA_WITH_(?:3DES_EDE_CBC_SHA|AES_(?:128_(?:CBC_SHA(?:256)?|GCM_SHA256)|256_(?:CBC_SHA(?:384)?|GCM_SHA384))|CHACHA20_POLY1305_SHA256))|RSA_WITH_(?:3DES_EDE_CBC_SHA|AES_(?:128_(?:CBC_SHA(?:256)?|GCM_SHA256)|256_(?:CBC_SHA(?:256)?|GCM_SHA384)))|group_(?:ats(?:_compatibility)?|compatibility|default|legacy))|protocol_version_(?:DTLSv1(?:0|2)|TLSv1(?:0|1|2|3)))|m(?:foErr|wdoErr)|o(?:g(?:Char(?:12HourBit|ZCycleBit)|Delta12HourBit|gle(?:B(?:ad(?:Char|Delta|Field|Num)|it)|Err(?:3|4|5)|O(?:K|utOfRange)|Un(?:defined|known)))|k(?:DecPoint|E(?:Minus|Plus|scape)|Le(?:ad(?:Placer|er)|ftQuote)|M(?:axSymbols|inusSign)|NonLeader|P(?:ercent|lusSign)|R(?:eserved|ightQuote)|Separator|Thousands|ZeroLead|en(?:1Quote|2(?:Equal|Quote)|A(?:l(?:pha|t(?:Num|Real))|mpersand|sterisk|tSign)|Ba(?:ckSlash|r)|C(?:a(?:pPi|r(?:at|et))|enterDot|o(?:lon(?:Equal)?|mma))|D(?:ivide|ollar)|E(?:llipsis|mpty|qual|rr|scape|xclam(?:Equal)?)|Fraction|Great(?:Equal(?:1|2))?|Hash|In(?:finity|t(?:egral|l(?:Currency)?))|L(?:e(?:ft(?:1Quote|2Quote|Bracket|C(?:omment|urly)|Enclose|Lit|Paren|SingGuillemet)|ss(?:Equal(?:1|2)|Great)?)|iteral)|Mi(?:cro|nus)|N(?:ewLine|il|o(?:BreakSpace|tEqual)|umeric)|O(?:K|verflow)|P(?:er(?:Thousand|cent|iod)|i|lus(?:Minus)?)|Question|R(?:e(?:alNum|serve(?:1|2))|ight(?:1Quote|2Quote|Bracket|C(?:omment|urly)|Enclose|Lit|Paren|SingGuillemet)|oot)|S(?:emicolon|igma|lash)|Tild(?:a|e)|Un(?:derline|known)|White))|oMany(?:Reqs|S(?:eps|kts)))|ra(?:ck(?:IDNotFound|NotInMovie)|shIconResource)|s(?:N(?:extSelectMode|ormalSelectMode)|PreviousSelectMode|m(?:AlreadyRegisteredErr|C(?:ant(?:ChangeForcedClassStateErr|OpenComponentErr)|omponent(?:AlreadyOpenErr|NoErr|Property(?:NotFoundErr|UnsupportedErr)))|D(?:efaultIsNotInputMethodErr|oc(?:NotActiveErr|Property(?:BufferTooSmallErr|NotFoundErr)|umentOpenErr))|In(?:putM(?:ethod(?:IsOldErr|NotFoundErr)|odeChangeFailedErr)|valid(?:Context|DocIDErr))|N(?:everRegisteredErr|o(?:Handler|MoreTokens|OpenTSErr|Stem|tAnAppErr))|ScriptHasNoIMErr|T(?:S(?:HasNoMenuErr|MDocBusyErr|NotOpenErr)|extServiceNotFoundErr)|U(?:n(?:knownErr|sup(?:ScriptLanguageErr|portedTypeErr))|seInputWindowErr)))|t(?:Disabled|Label(?:1|2|3|4|5|6|7)|None|O(?:ffline|pen)|Selected(?:Disabled|O(?:ffline|pen))?)|uneP(?:arseOSErr|layerFullOSErr)|woSideErr|ype(?:128BitFloatingPoint|32BitIcon|4BitIcon|8Bit(?:Icon|Mask)|A(?:E(?:Homograph(?:Accent|DicInfo|Weight)|List|Morpheme(?:PartOfSpeechCode|TextRange)|Record|T(?:E|ext)|UT)|SStorage|TS(?:FontRef|U(?:FontID|Size))|bsoluteOrdinal|lias|pp(?:Parameters|l(?:Signature|e(?:Event|Script)|ication(?:BundleID|URL)))|rc|uditToken)|B(?:est|oo(?:kmarkData|lean)|yte(?:Count|Offset))|C(?:F(?:A(?:bsoluteTime|rrayRef|ttributedStringRef)|BooleanRef|DictionaryRef|Index|Mutable(?:A(?:rrayRef|ttributedStringRef)|DictionaryRef|StringRef)|NumberRef|Range|StringRef|TypeRef)|G(?:ContextRef|Display(?:ChangeFlags|ID)|Float(?:72DPIGlobal|ScreenPixel)?|ImageRef)|String|T(?:Font(?:DescriptorRef|Ref)|GlyphInfoRef)|e(?:ll|ntimeters)|har|l(?:assInfo|ickActivationResult)|o(?:l(?:lection|orTable|umn)|mp(?:Descriptor|onentInstance)|n(?:ceptualTime|trol(?:ActionUPP|FrameMetrics|PartCode|Ref)))|u(?:bic(?:Centimeter|Feet|Inches|Meters|Yards)|rrentContainer))|D(?:CMFi(?:eldAttributes|ndMethod)|a(?:shStyle|ta)|e(?:cimalStruct|grees(?:C|F|K))|ra(?:gRef|wingArea))|E(?:PS|lemInfo|n(?:codedString|umerat(?:ed|ion))|vent(?:HotKeyID|Info|Re(?:cord|f)|Target(?:Options|Ref)))|F(?:MFont(?:Family|S(?:ize|tyle))|S(?:Ref|VolumeRefNum)|alse|eet|i(?:leURL|nderWindow|xed(?:Point|Rectangle)?)|ontColor)|G(?:DHandle|IF|WorldPtr|allons|lyph(?:InfoArray|Selector)|r(?:a(?:fPtr|ms|phic(?:Line|Text))|oupedGraphic))|HI(?:Command|Menu|ObjectRef|Point(?:72DPIGlobal|ScreenPixel)?|Rect(?:72DPIGlobal|ScreenPixel)?|S(?:hapeRef|ize(?:72DPIGlobal|ScreenPixel)?)|Toolbar(?:Display(?:Mode|Size)|ItemRef|Ref)|ViewTrackingAreaRef|Window)|I(?:EEE(?:32BitFloatingPoint|64BitFloatingPoint)|SO8601DateTime|con(?:AndMask|Family)|n(?:ches|d(?:exDescriptor|icatorDragConstraint)|sertionLoc|tl(?:Text|WritingCode)))|JPEG|K(?:e(?:rnelProcessID|yword)|ilo(?:grams|meters))|L(?:A(?:Homograph|Morpheme(?:Bundle|Path)?)|iters|o(?:gicalDescriptor|ng(?:DateTime|Fixed(?:Point|Rectangle)?|Point|Rectangle)|wLevelEventRecord))|M(?:ach(?:Port|ineLoc)|e(?:nu(?:Command|Direction|EventOptions|ItemIndex|Ref|TrackingMode)|ters)|iles|o(?:dalClickResult|use(?:Button|TrackingRef|WheelAxis)))|Null|O(?:S(?:A(?:DialectInfo|ErrorRange|GenericStorage)|LTokenList|Status)|bject(?:BeingExamined|Specifier)|ffsetArray|unces|val)|P(?:String|a(?:ramInfo|steboardRef)|i(?:ct|x(?:MapMinus|elMap))|o(?:lygon|unds)|ro(?:cessSerialNumber|p(?:Info|erty))|tr)|Q(?:D(?:Point|R(?:e(?:ctangle|gion)|gnHandle))|uarts)|R(?:GB(?:16|96|Color)|angeDescriptor|e(?:ctangle|fCon|lative(?:Descriptor|Time)|plyPortAttr)|o(?:tation|undedRectangle|w))|S(?:Int(?:16|32|64)|R(?:Recognizer|SpeechResult)|c(?:r(?:ap(?:Ref|Styles)|ipt)|szResource)|ec(?:IdentityRef|tionH)|ignedByte(?:Count|Offset)|mall(?:32BitIcon|4BitIcon|8Bit(?:Icon|Mask)|IconAndMask)|ound|quare(?:Feet|Kilometers|M(?:eters|iles)|Yards)|tyled(?:Text|UnicodeText)|uiteInfo)|T(?:IFF|able(?:tP(?:oint(?:Rec|erRec)|roximityRec))?|ext(?:Range(?:Array)?|Styles)?|hemeMenu(?:ItemType|State|Type)|oken|rue|ype)|U(?:Int(?:16|32|64)|TF(?:16ExternalRepresentation|8Text)|nicodeText|serRecordFields)|V(?:ersion|oidPtr)|W(?:hose(?:Descriptor|Range)|i(?:ldCard|ndow(?:DefPartCode|Modality|PartCode|Re(?:f|gionCode)|Transition(?:Action|Effect))))|Yards))|u(?:n(?:doDev|i(?:code(?:BufErr|C(?:h(?:arErr|ecksumErr)|ontextualErr)|DirectionErr|ElementErr|FallbacksErr|No(?:TableErr|tFoundErr)|PartConvertErr|T(?:ableFormatErr|extEncodingDataErr)|VariantErr)|mpErr|t(?:EmptyErr|TblFullErr))|known(?:FormatErr|InsertModeErr)|resolvedComponentDLLErr|supported(?:AuxiliaryImportData|ForPlatformErr|OSErr|ProcessorErr))|p(?:d(?:PixMemErr|ate(?:Dev|Evt|Mask))|p(?:C(?:allComponent(?:C(?:anDoProcInfo|loseProcInfo)|Get(?:MPWorkFunctionProcInfo|PublicResourceProcInfo)|OpenProcInfo|RegisterProcInfo|TargetProcInfo|UnregisterProcInfo|VersionProcInfo)|omponent(?:FunctionImplementedProcInfo|SetTargetProcInfo))|GetComponentVersionProcInfo))|rlDataH(?:FTP(?:Bad(?:NameListErr|PasswordErr|UserErr)|DataConnectionErr|FilenameErr|N(?:eedPasswordErr|o(?:DirectoryErr|NetDriverErr|PasswordErr))|P(?:ermissionsErr|rotocolErr)|QuotaErr|S(?:erver(?:DisconnectedErr|Err)|hutdownErr)|URLErr)|HTTP(?:NoNetDriverErr|ProtocolErr|RedirectErr|URLErr))|se(?:A(?:Talk|sync)|ExtClk|Free|MIDI|r(?:Break|CanceledErr|DataItemNotFound|Item|Kind|RejectErr)))|v(?:AxisOnly|LckdErr|Typ(?:Err|e)|a(?:lid(?:DateFields|InstancesExist)|riationFontTableTag)|er(?:A(?:frikaans|lternateGr|r(?:abi(?:a|c)|menia(?:n)?)|ustr(?:alia|ia(?:German)?))|B(?:e(?:l(?:arus|giumLux(?:Point)?)|ngali)|hutan|r(?:azil|eton|it(?:ain|tany))|ulgaria|yeloRussian)|C(?:a(?:nada(?:Comma|Point)|talonia)|hina|roatia|yprus|zech)|Denmark|E(?:astAsiaGeneric|ngCanada|rr|s(?:peranto|tonia))|F(?:a(?:eroeIsl|r(?:EastGeneric|oeIsl))|inland|lemish(?:Point)?|r(?:Belgium(?:Lux)?|Canada|Swiss|ance|enchUniversal))|G(?:e(?:nericFE|orgia(?:n)?|rman(?:Reformed|y))|r(?:Swiss|ee(?:ce(?:Alt|Poly)?|kAncient|nland))|ujarati)|Hungary|I(?:celand|n(?:dia(?:Hindi|Urdu)?|ternational)|r(?:an|eland(?:English)?|ishGaelicScript)|srael|tal(?:ianSwiss|y))|Japan|Korea|L(?:a(?:pland|tvia)|ithuania)|M(?:a(?:cedonia(?:n)?|gyar|lta|nxGaelic|rathi)|ultilingual)|N(?:e(?:pal|therlands(?:Comma)?)|orway|unavut|ynorsk)|P(?:akistan(?:Urdu)?|o(?:land|rtugal)|unjabi)|R(?:omania|u(?:mania|ssia))|S(?:ami|c(?:ottishGaelic|riptGeneric)|erbia(?:n)?|ingapore|lov(?:ak|enia(?:n)?)|p(?:LatinAmerica|ain)|weden)|T(?:aiwan|hailand|ibet(?:an)?|onga|urk(?:ey|ishModified))|U(?:S|kra(?:ine|nia)|nspecified|zbek)|Vietnam|W(?:ales|elsh)|Yugo(?:Croatian|slavia)|variant(?:Denmark|Norway|Portugal))|ideoOutputInUseErr|m(?:AddressNotInFileViewErr|B(?:adDriver|usyBackingFileErr)|FileViewAccessErr|Invalid(?:BackingFileIDErr|FileViewIDErr|OwningProcessErr)|KernelMMUInitErr|M(?:appingPrivilegesErr|emLckdErr|orePhysicalThanVirtualErr)|No(?:More(?:BackingFilesErr|FileViewsErr)|VectorErr)|OffErr)|o(?:iceNotFound|l(?:GoneErr|Mount(?:Changed(?:Bit|Mask)|ExtendedFlags(?:Bit|Mask)|FSReservedMask|Interact(?:Bit|Mask)|NoLoginMsgFlag(?:Bit|Mask)|SysReservedMask)|O(?:ffLinErr|nLinErr)|VMBusyErr)))|w(?:C(?:alcRgns|ontentColor)|D(?:ispose|raw(?:GIcon)?)|FrameColor|Grow|Hi(?:liteColor|t)|In(?:Co(?:llapseBox|ntent)|Drag|G(?:oAway|row)|ProxyIcon|Structure|ToolbarButton|Zoom(?:In|Out))|N(?:ew|oHit)|PrErr|T(?:extColor|itleBarColor)|ack(?:Bad(?:FileErr|MetaDataErr)|ForkNotFoundErr)|eekOfYear(?:Field|Mask)|fFileNotFound|indow(?:A(?:ppModalStateAlreadyExistsErr|ttribute(?:ImmutableErr|sConflictErr))|GroupInvalidErr|ManagerInternalErr|NoAppModalStateErr|WrongStateErr)|r(?:PermErr|Underrun|gVolTypErr|it(?:Err|eReference|ingPastEnd)|ongApplicationPlatform))|y(?:dm|ear(?:Field|Mask)|md)|z(?:eroCycle|oom(?:DocProc|NoGrow)))\\b",
+ "name": "support.constant.c"
+ },
{
"match": "\\bkCFNumberFormatter(?:Currency(?:AccountingStyle|ISOCodeStyle|PluralStyle)|OrdinalStyle)\\b",
"name": "support.constant.cf.10.11.c"
@@ -57,6 +209,14 @@
"match": "\\bkCFISO8601DateFormatWith(?:ColonSeparatorInTime(?:Zone)?|Da(?:shSeparatorInDate|y)|Full(?:Date|Time)|InternetDateTime|Month|SpaceBetweenDateAndTime|Time(?:Zone)?|WeekOfYear|Year)\\b",
"name": "support.constant.cf.10.12.c"
},
+ {
+ "match": "\\bkCFISO8601DateFormatWithFractionalSeconds\\b",
+ "name": "support.constant.cf.10.13.c"
+ },
+ {
+ "match": "\\bkCFURLEnumeratorGenerateRelativePathURLs\\b",
+ "name": "support.constant.cf.10.15.c"
+ },
{
"match": "\\bkCFFileSecurityClear(?:AccessControlList|Group(?:UUID)?|Mode|Owner(?:UUID)?)\\b",
"name": "support.constant.cf.10.8.c"
@@ -65,6 +225,10 @@
"match": "\\b(?:CF(?:ByteOrder(?:BigEndian|LittleEndian|Unknown)|NotificationSuspensionBehavior(?:Coalesce|D(?:eliverImmediately|rop)|Hold))|kCF(?:B(?:ookmarkResolutionWithout(?:MountingMask|UIMask)|undleExecutableArchitecture(?:I386|PPC(?:64)?|X86_64))|C(?:alendar(?:ComponentsWrap|Unit(?:Day|Era|Hour|M(?:inute|onth)|Quarter|Second|Week(?:Of(?:Month|Year)|day(?:Ordinal)?)|Year(?:ForWeekOfYear)?))|haracterSet(?:AlphaNumeric|C(?:apitalizedLetter|ontrol)|Dec(?:imalDigit|omposable)|Illegal|L(?:etter|owercaseLetter)|N(?:ewline|onBase)|Punctuation|Symbol|UppercaseLetter|Whitespace(?:AndNewline)?)|ompare(?:Anchored|Backwards|CaseInsensitive|DiacriticInsensitive|EqualTo|ForcedOrdering|GreaterThan|L(?:essThan|ocalized)|N(?:onliteral|umerically)|WidthInsensitive))|Dat(?:aSearch(?:Anchored|Backwards)|eFormatter(?:FullStyle|LongStyle|MediumStyle|NoStyle|ShortStyle))|FileDescriptor(?:ReadCallBack|WriteCallBack)|LocaleLanguageDirection(?:BottomToTop|LeftToRight|RightToLeft|TopToBottom|Unknown)|MessagePort(?:BecameInvalidError|IsInvalid|ReceiveTimeout|S(?:endTimeout|uccess)|TransportError)|N(?:otification(?:DeliverImmediately|PostToAllSessions)|umber(?:C(?:FIndexType|GFloatType|harType)|DoubleType|F(?:loat(?:32Type|64Type|Type)|ormatter(?:CurrencyStyle|DecimalStyle|NoStyle|P(?:a(?:d(?:After(?:Prefix|Suffix)|Before(?:Prefix|Suffix))|rseIntegersOnly)|ercentStyle)|Round(?:Ceiling|Down|Floor|Half(?:Down|Even|Up)|Up)|S(?:cientificStyle|pellOutStyle)))|IntType|Long(?:LongType|Type)|MaxType|NSIntegerType|S(?:Int(?:16Type|32Type|64Type|8Type)|hortType)))|PropertyList(?:BinaryFormat_v1_0|Immutable|MutableContainers(?:AndLeaves)?|OpenStepFormat|Read(?:CorruptError|StreamError|UnknownVersionError)|WriteStreamError|XMLFormat_v1_0)|RunLoop(?:A(?:fterWaiting|llActivities)|Before(?:Sources|Timers|Waiting)|E(?:ntry|xit)|Run(?:Finished|HandledSource|Stopped|TimedOut))|S(?:ocket(?:A(?:cceptCallBack|utomaticallyReenable(?:AcceptCallBack|DataCallBack|ReadCallBack|WriteCallBack))|C(?:loseOnInvalidate|onnectCallBack)|DataCallBack|Error|LeaveErrors|NoCallBack|ReadCallBack|Success|Timeout|WriteCallBack)|tr(?:eam(?:E(?:rrorDomain(?:Custom|MacOSStatus|POSIX)|vent(?:CanAcceptBytes|E(?:ndEncountered|rrorOccurred)|HasBytesAvailable|None|OpenCompleted))|Status(?:AtEnd|Closed|Error|NotOpen|Open(?:ing)?|Reading|Writing))|ing(?:Encoding(?:A(?:NSEL|SCII)|Big5(?:_(?:E|HKSCS_1999))?|CNS_11643_92_P(?:1|2|3)|DOS(?:Arabic|BalticRim|C(?:anadianFrench|hinese(?:Simplif|Trad)|yrillic)|Greek(?:1|2)?|Hebrew|Icelandic|Japanese|Korean|Latin(?:1|2|US)|Nordic|Portuguese|Russian|T(?:hai|urkish))|E(?:BCDIC_(?:CP037|US)|UC_(?:CN|JP|KR|TW))|GB(?:K_95|_(?:18030_2000|2312_80))|HZ_GB_2312|ISO(?:Latin(?:1(?:0)?|2|3|4|5|6|7|8|9|Arabic|Cyrillic|Greek|Hebrew|Thai)|_2022_(?:CN(?:_EXT)?|JP(?:_(?:1|2|3))?|KR))|JIS_(?:C6226_78|X02(?:0(?:1_76|8_(?:83|90))|12_90))|K(?:OI8_(?:R|U)|SC_5601_(?:87|92_Johab))|Mac(?:Ar(?:abic|menian)|B(?:engali|urmese)|C(?:e(?:ltic|ntralEurRoman)|hinese(?:Simp|Trad)|roatian|yrillic)|D(?:evanagari|ingbats)|E(?:thiopic|xtArabic)|Farsi|G(?:aelic|eorgian|reek|u(?:jarati|rmukhi))|H(?:FS|ebrew)|I(?:celandic|nuit)|Japanese|K(?:annada|hmer|orean)|Laotian|M(?:alayalam|ongolian)|Oriya|Roman(?:Latin1|ian)?|S(?:inhalese|ymbol)|T(?:amil|elugu|hai|ibetan|urkish)|Ukrainian|V(?:T100|ietnamese))|N(?:extStep(?:Japanese|Latin)|onLossyASCII)|ShiftJIS(?:_X0213(?:_(?:00|MenKuTen))?)?|U(?:TF(?:16(?:BE|LE)?|32(?:BE|LE)?|7(?:_IMAP)?|8)|nicode)|VISCII|Windows(?:Arabic|BalticRim|Cyrillic|Greek|Hebrew|KoreanJohab|Latin(?:1|2|5)|Vietnamese))|NormalizationForm(?:C|D|K(?:C|D))|Tokenizer(?:AttributeLa(?:nguage|tinTranscription)|Token(?:Has(?:DerivedSubTokensMask|HasNumbersMask|NonLettersMask|SubTokensMask)|IsCJWordMask|No(?:ne|rmal))|Unit(?:LineBreak|Paragraph|Sentence|Word(?:Boundary)?)))))|TimeZoneNameStyle(?:DaylightSaving|Generic|S(?:hort(?:DaylightSaving|Generic|Standard)|tandard))|U(?:RL(?:Bookmark(?:Creation(?:MinimalBookmarkMask|S(?:ecurityScopeAllowOnlyReadAccess|uitableForBookmarkFile)|WithSecurityScope)|ResolutionWith(?:SecurityScope|out(?:MountingMask|UIMask)))|Component(?:Fragment|Host|NetLocation|P(?:a(?:rameterString|ssword|th)|ort)|Query|ResourceSpecifier|Scheme|User(?:Info)?)|Enumerator(?:D(?:e(?:faultBehavior|scendRecursively)|irectoryPostOrderSuccess)|E(?:nd|rror)|GenerateFileReferenceURLs|IncludeDirectoriesP(?:ostOrder|reOrder)|S(?:kip(?:Invisibles|PackageContents)|uccess))|POSIXPathStyle|WindowsPathStyle)|serNotification(?:AlternateResponse|Ca(?:ncelResponse|utionAlertLevel)|DefaultResponse|No(?:DefaultButtonFlag|teAlertLevel)|OtherResponse|PlainAlertLevel|StopAlertLevel|UseRadioButtonsFlag))|XML(?:E(?:ntityType(?:Character|Par(?:ameter|sed(?:External|Internal))|Unparsed)|rror(?:E(?:lementlessDocument|ncodingConversionFailure)|Malformed(?:C(?:DSect|haracterReference|loseTag|omment)|D(?:TD|ocument)|Name|P(?:arsedCharacterData|rocessingInstruction)|StartTag)|NoData|Un(?:expectedEOF|knownEncoding)))|Node(?:CurrentVersion|Type(?:Attribute(?:ListDeclaration)?|C(?:DATASection|omment)|Document(?:Fragment|Type)?|E(?:lement(?:TypeDeclaration)?|ntity(?:Reference)?)|Notation|ProcessingInstruction|Text|Whitespace))|Parser(?:A(?:ddImpliedAttributes|llOptions)|NoOptions|Re(?:placePhysicalEntities|solveExternalEntities)|Skip(?:MetaData|Whitespace)|ValidateDocument)|StatusParse(?:InProgress|NotBegun|Successful))))\\b",
"name": "support.constant.cf.c"
},
+ {
+ "match": "\\bDISPATCH_WALLTIME_NOW\\b",
+ "name": "support.constant.clib.10.14.c"
+ },
{
"match": "\\b(?:FILESEC_(?:ACL(?:_(?:ALLOCSIZE|RAW))?|GR(?:OUP|PUUID)|MODE|OWNER|UUID)|P_(?:ALL|P(?:GID|ID)))\\b",
"name": "support.constant.clib.c"
@@ -86,23 +250,39 @@
"name": "support.constant.os.c"
},
{
- "match": "\\bkCGImageByteOrder(?:16(?:Big|Little)|32(?:Big|Little)|Mask)\\b",
- "name": "support.constant.quartz.10.12.c"
+ "match": "\\bkCGImagePixelFormat(?:Mask|Packed|RGB(?:101010|5(?:55|65)|CIF10))\\b",
+ "name": "support.constant.quartz.10.14.c"
+ },
+ {
+ "match": "\\bCGPDFTagType(?:A(?:nnotation|rt)|B(?:ibliography|lockQuote)|C(?:aption|ode)|D(?:iv|ocument)|F(?:igure|orm(?:ula)?)|Header(?:1|2|3|4|5|6)?|Index|L(?:abel|i(?:nk|st(?:Body|Item)?))|No(?:nStructure|te)|P(?:ar(?:agraph|t)|rivate)|Quote|R(?:eference|uby(?:AnnotationText|BaseText|Punctuation)?)|S(?:ection|pan)|T(?:OC(?:I)?|able(?:Body|DataCell|Footer|Header(?:Cell)?|Row)?)|Warichu(?:Punctiation|Text)?)\\b",
+ "name": "support.constant.quartz.10.15.c"
},
{
- "match": "\\b(?:CG(?:GlyphM(?:ax|in)|PDFDataFormat(?:JPEG(?:2000|Encoded)|Raw)|RectM(?:ax(?:XEdge|YEdge)|in(?:XEdge|YEdge)))|kCG(?:A(?:nnotatedSessionEventTap|ssistiveTechHighWindowLevelKey)|B(?:a(?:ck(?:ingStore(?:Buffered|Nonretained|Retained)|stopMenuLevelKey)|seWindowLevelKey)|itmap(?:AlphaInfoMask|ByteOrder(?:16(?:Big|Little)|32(?:Big|Little)|Default|Mask)|Float(?:Components|InfoMask))|lendMode(?:C(?:lear|o(?:lor(?:Burn|Dodge)?|py))|D(?:arken|estination(?:Atop|In|O(?:ut|ver))|ifference)|Exclusion|H(?:ardLight|ue)|L(?:ighten|uminosity)|Multiply|Normal|Overlay|Plus(?:Darker|Lighter)|S(?:aturation|creen|o(?:ftLight|urce(?:Atop|In|Out)))|XOR))|C(?:aptureNo(?:Fill|Options)|o(?:lor(?:ConversionTransform(?:ApplySpace|FromSpace|ToSpace)|SpaceModel(?:CMYK|DeviceN|Indexed|Lab|Monochrome|Pattern|RGB|Unknown))|nfigure(?:For(?:AppOnly|Session)|Permanently))|ursorWindowLevelKey)|D(?:esktop(?:IconWindowLevelKey|WindowLevelKey)|isplay(?:AddFlag|BeginConfigurationFlag|D(?:esktopShapeChangedFlag|isabledFlag)|EnabledFlag|M(?:irrorFlag|ovedFlag)|RemoveFlag|S(?:etM(?:ainFlag|odeFlag)|tream(?:FrameStatus(?:Frame(?:Blank|Complete|Idle)|Stopped)|Update(?:DirtyRects|MovedRects|Re(?:ducedDirtyRects|freshedRects))))|UnMirrorFlag)|ockWindowLevelKey|raggingWindowLevelKey)|E(?:rror(?:CannotComplete|Failure|I(?:llegalArgument|nvalid(?:Con(?:nection|text)|Operation))|No(?:neAvailable|tImplemented)|RangeCheck|Success|TypeCheck)|vent(?:F(?:ilterMaskPermit(?:Local(?:KeyboardEvents|MouseEvents)|SystemDefinedEvents)|lag(?:Mask(?:Al(?:phaShift|ternate)|Co(?:mmand|ntrol)|Help|N(?:onCoalesced|umericPad)|S(?:econdaryFn|hift))|sChanged))|Key(?:Down|Up)|LeftMouse(?:D(?:own|ragged)|Up)|Mouse(?:Moved|Subtype(?:Default|TabletP(?:oint|roximity)))|Null|OtherMouse(?:D(?:own|ragged)|Up)|RightMouse(?:D(?:own|ragged)|Up)|S(?:crollWheel|ource(?:GroupID|State(?:CombinedSessionState|HIDSystemState|ID|Private)|U(?:nixProcessID|ser(?:Data|ID)))|uppressionState(?:RemoteMouseDrag|SuppressionInterval))|Ta(?:bletP(?:ointer|roximity)|p(?:DisabledBy(?:Timeout|UserInput)|Option(?:Default|ListenOnly))|rget(?:ProcessSerialNumber|UnixProcessID))))|F(?:loatingWindowLevelKey|ontPostScriptFormatType(?:1|3|42))|G(?:esturePhase(?:Began|C(?:ancelled|hanged)|Ended|MayBegin|None)|radientDraws(?:AfterEndLocation|BeforeStartLocation))|H(?:IDEventTap|e(?:adInsertEventTap|lpWindowLevelKey))|I(?:mageAlpha(?:First|Last|None(?:Skip(?:First|Last))?|Only|Premultiplied(?:First|Last))|nterpolation(?:Default|High|Low|Medium|None))|KeyboardEvent(?:Autorepeat|Key(?:boardType|code))|Line(?:Cap(?:Butt|Round|Square)|Join(?:Bevel|Miter|Round))|M(?:a(?:inMenuWindowLevelKey|ximumWindowLevelKey)|inimumWindowLevelKey|o(?:dalPanelWindowLevelKey|mentumScrollPhase(?:Begin|Continue|End|None)|use(?:Button(?:Center|Left|Right)|Event(?:ButtonNumber|ClickState|Delta(?:X|Y)|InstantMouser|Number|Pressure|Subtype|WindowUnderMousePointer(?:ThatCanHandleThisEvent)?))))|N(?:ormalWindowLevelKey|umberOf(?:EventSuppressionStates|WindowLevelKeys))|OverlayWindowLevelKey|P(?:DF(?:ArtBox|BleedBox|CropBox|MediaBox|ObjectType(?:Array|Boolean|Dictionary|Integer|N(?:ame|ull)|Real|Str(?:eam|ing))|TrimBox)|at(?:h(?:E(?:OFill(?:Stroke)?|lement(?:Add(?:CurveToPoint|LineToPoint|QuadCurveToPoint)|CloseSubpath|MoveToPoint))|Fill(?:Stroke)?|Stroke)|ternTiling(?:ConstantSpacing(?:MinimalDistortion)?|NoDistortion))|opUpMenuWindowLevelKey)|RenderingIntent(?:AbsoluteColorimetric|Default|Perceptual|RelativeColorimetric|Saturation)|S(?:cr(?:een(?:SaverWindowLevelKey|UpdateOperation(?:Move|Re(?:ducedDirtyRectangleCount|fresh)))|oll(?:EventUnit(?:Line|Pixel)|Phase(?:Began|C(?:ancelled|hanged)|Ended|MayBegin)|WheelEvent(?:DeltaAxis(?:1|2|3)|FixedPtDeltaAxis(?:1|2|3)|I(?:nstantMouser|sContinuous)|MomentumPhase|PointDeltaAxis(?:1|2|3)|Scroll(?:Count|Phase))))|essionEventTap|tatusWindowLevelKey)|T(?:a(?:blet(?:Event(?:DeviceID|Point(?:Buttons|Pressure|X|Y|Z)|Rotation|T(?:angentialPressure|ilt(?:X|Y))|Vendor(?:1|2|3))|ProximityEvent(?:CapabilityMask|DeviceID|EnterProximity|Pointer(?:ID|Type)|SystemTabletID|TabletID|Vendor(?:ID|Pointer(?:SerialNumber|Type)|UniqueID)))|ilAppendEventTap)|ext(?:Clip|Fill(?:Clip|Stroke(?:Clip)?)?|Invisible|Stroke(?:Clip)?)|ornOffMenuWindowLevelKey)|UtilityWindowLevelKey|Window(?:Image(?:B(?:estResolution|oundsIgnoreFraming)|Default|NominalResolution|OnlyShadows|ShouldBeOpaque)|List(?:ExcludeDesktopElements|Option(?:All|IncludingWindow|OnScreen(?:AboveWindow|BelowWindow|Only)))|Sharing(?:None|Read(?:Only|Write)))))\\b",
+ "match": "\\b(?:CG(?:GlyphM(?:ax|in)|PDFDataFormat(?:JPEG(?:2000|Encoded)|Raw)|RectM(?:ax(?:XEdge|YEdge)|in(?:XEdge|YEdge)))|kCG(?:A(?:nnotatedSessionEventTap|ssistiveTechHighWindowLevelKey)|B(?:a(?:ck(?:ingStore(?:Buffered|Nonretained|Retained)|stopMenuLevelKey)|seWindowLevelKey)|itmap(?:AlphaInfoMask|ByteOrder(?:16(?:Big|Little)|32(?:Big|Little)|Default|Mask)|Float(?:Components|InfoMask))|lendMode(?:C(?:lear|o(?:lor(?:Burn|Dodge)?|py))|D(?:arken|estination(?:Atop|In|O(?:ut|ver))|ifference)|Exclusion|H(?:ardLight|ue)|L(?:ighten|uminosity)|Multiply|Normal|Overlay|Plus(?:Darker|Lighter)|S(?:aturation|creen|o(?:ftLight|urce(?:Atop|In|Out)))|XOR))|C(?:aptureNo(?:Fill|Options)|o(?:lor(?:ConversionTransform(?:ApplySpace|FromSpace|ToSpace)|SpaceModel(?:CMYK|DeviceN|Indexed|Lab|Monochrome|Pattern|RGB|Unknown|XYZ))|nfigure(?:For(?:AppOnly|Session)|Permanently))|ursorWindowLevelKey)|D(?:esktop(?:IconWindowLevelKey|WindowLevelKey)|isplay(?:AddFlag|BeginConfigurationFlag|D(?:esktopShapeChangedFlag|isabledFlag)|EnabledFlag|M(?:irrorFlag|ovedFlag)|RemoveFlag|S(?:etM(?:ainFlag|odeFlag)|tream(?:FrameStatus(?:Frame(?:Blank|Complete|Idle)|Stopped)|Update(?:DirtyRects|MovedRects|Re(?:ducedDirtyRects|freshedRects))))|UnMirrorFlag)|ockWindowLevelKey|raggingWindowLevelKey)|E(?:rror(?:CannotComplete|Failure|I(?:llegalArgument|nvalid(?:Con(?:nection|text)|Operation))|No(?:neAvailable|tImplemented)|RangeCheck|Success|TypeCheck)|vent(?:F(?:ilterMaskPermit(?:Local(?:KeyboardEvents|MouseEvents)|SystemDefinedEvents)|lag(?:Mask(?:Al(?:phaShift|ternate)|Co(?:mmand|ntrol)|Help|N(?:onCoalesced|umericPad)|S(?:econdaryFn|hift))|sChanged))|Key(?:Down|Up)|LeftMouse(?:D(?:own|ragged)|Up)|Mouse(?:Moved|Subtype(?:Default|TabletP(?:oint|roximity)))|Null|OtherMouse(?:D(?:own|ragged)|Up)|RightMouse(?:D(?:own|ragged)|Up)|S(?:crollWheel|ource(?:GroupID|State(?:CombinedSessionState|HIDSystemState|ID|Private)|U(?:nixProcessID|ser(?:Data|ID)))|uppressionState(?:RemoteMouseDrag|SuppressionInterval))|Ta(?:bletP(?:ointer|roximity)|p(?:DisabledBy(?:Timeout|UserInput)|Option(?:Default|ListenOnly))|rget(?:ProcessSerialNumber|UnixProcessID))|UnacceleratedPointerMovement(?:X|Y)))|F(?:loatingWindowLevelKey|ontPostScriptFormatType(?:1|3|42))|G(?:esturePhase(?:Began|C(?:ancelled|hanged)|Ended|MayBegin|None)|radientDraws(?:AfterEndLocation|BeforeStartLocation))|H(?:IDEventTap|e(?:adInsertEventTap|lpWindowLevelKey))|I(?:mage(?:Alpha(?:First|Last|None(?:Skip(?:First|Last))?|Only|Premultiplied(?:First|Last))|ByteOrder(?:16(?:Big|Little)|32(?:Big|Little)|Default|Mask))|nterpolation(?:Default|High|Low|Medium|None))|KeyboardEvent(?:Autorepeat|Key(?:boardType|code))|Line(?:Cap(?:Butt|Round|Square)|Join(?:Bevel|Miter|Round))|M(?:a(?:inMenuWindowLevelKey|ximumWindowLevelKey)|inimumWindowLevelKey|o(?:dalPanelWindowLevelKey|mentumScrollPhase(?:Begin|Continue|End|None)|use(?:Button(?:Center|Left|Right)|Event(?:ButtonNumber|ClickState|Delta(?:X|Y)|InstantMouser|Number|Pressure|Subtype|WindowUnderMousePointer(?:ThatCanHandleThisEvent)?))))|N(?:ormalWindowLevelKey|umberOf(?:EventSuppressionStates|WindowLevelKeys))|OverlayWindowLevelKey|P(?:DF(?:A(?:llows(?:Co(?:mmenting|ntent(?:Accessibility|Copying))|Document(?:Assembly|Changes)|FormFieldEntry|HighQualityPrinting|LowQualityPrinting)|rtBox)|BleedBox|CropBox|MediaBox|ObjectType(?:Array|Boolean|Dictionary|Integer|N(?:ame|ull)|Real|Str(?:eam|ing))|TrimBox)|at(?:h(?:E(?:OFill(?:Stroke)?|lement(?:Add(?:CurveToPoint|LineToPoint|QuadCurveToPoint)|CloseSubpath|MoveToPoint))|Fill(?:Stroke)?|Stroke)|ternTiling(?:ConstantSpacing(?:MinimalDistortion)?|NoDistortion))|opUpMenuWindowLevelKey)|RenderingIntent(?:AbsoluteColorimetric|Default|Perceptual|RelativeColorimetric|Saturation)|S(?:cr(?:een(?:SaverWindowLevelKey|UpdateOperation(?:Move|Re(?:ducedDirtyRectangleCount|fresh)))|oll(?:EventUnit(?:Line|Pixel)|Phase(?:Began|C(?:ancelled|hanged)|Ended|MayBegin)|WheelEvent(?:DeltaAxis(?:1|2|3)|FixedPtDeltaAxis(?:1|2|3)|I(?:nstantMouser|sContinuous)|MomentumPhase|PointDeltaAxis(?:1|2|3)|Scroll(?:Count|Phase))))|essionEventTap|tatusWindowLevelKey)|T(?:a(?:blet(?:Event(?:DeviceID|Point(?:Buttons|Pressure|X|Y|Z)|Rotation|T(?:angentialPressure|ilt(?:X|Y))|Vendor(?:1|2|3))|ProximityEvent(?:CapabilityMask|DeviceID|EnterProximity|Pointer(?:ID|Type)|SystemTabletID|TabletID|Vendor(?:ID|Pointer(?:SerialNumber|Type)|UniqueID)))|ilAppendEventTap)|ext(?:Clip|Fill(?:Clip|Stroke(?:Clip)?)?|Invisible|Stroke(?:Clip)?)|ornOffMenuWindowLevelKey)|UtilityWindowLevelKey|Window(?:Image(?:B(?:estResolution|oundsIgnoreFraming)|Default|NominalResolution|OnlyShadows|ShouldBeOpaque)|List(?:ExcludeDesktopElements|Option(?:All|IncludingWindow|OnScreen(?:AboveWindow|BelowWindow|Only)))|Sharing(?:None|Read(?:Only|Write)))))\\b",
"name": "support.constant.quartz.c"
},
+ {
+ "match": "\\bcl_device_id\\b",
+ "name": "support.type.10.10.c"
+ },
+ {
+ "match": "\\b(?:JSTypedArrayType|SecKey(?:Algorithm|KeyExchangeParameter)|os_unfair_lock(?:_t)?)\\b",
+ "name": "support.type.10.12.c"
+ },
+ {
+ "match": "\\b(?:A(?:E(?:A(?:ddressDesc|rray(?:Data(?:Pointer)?|Type))|BuildError(?:Code)?|Coerc(?:e(?:Desc(?:ProcPtr|UPP)|Ptr(?:ProcPtr|UPP))|ionHandlerUPP)|D(?:ataStorage(?:Type)?|esc(?:List|Ptr)?|isposeExternal(?:ProcPtr|UPP))|Event(?:Class|Handler(?:ProcPtr|UPP)|ID|Source)|Filter(?:ProcPtr|UPP)|I(?:dle(?:ProcPtr|UPP)|nteractAllowed)|Key(?:Desc|word)|Re(?:cord|moteProcessResolver(?:C(?:allback|ontext)|Ref)?|turnID)|S(?:end(?:Mode|Priority)|treamRef)|TransactionID)|FP(?:AlternateAddress|ServerSignature|TagData|VolMountInfo(?:Ptr)?|XVolMountInfo(?:Ptr)?)|HTOCType|IFFLoop|LMX(?:GlyphEntry|Header)|TS(?:Cu(?:bic(?:C(?:losePath(?:ProcPtr|UPP)|urveTo(?:ProcPtr|UPP))|LineTo(?:ProcPtr|UPP)|MoveTo(?:ProcPtr|UPP))|rveType)|F(?:SSpec|latData(?:Font(?:NameDataHeader|Spec(?:RawNameData(?:Header)?|iferType))|L(?:ayoutControlsDataHeader|ineInfo(?:Data|Header))|MainHeaderBlock|Style(?:List(?:FeatureData|Header|StyleDataHeader|VariationData)|RunDataHeader)|TextLayout(?:DataHeader|Header))|ont(?:A(?:pplierFunction|utoActivationSetting)|Cont(?:ainerRef|ext)|F(?:amily(?:ApplierFunction|Iterator(?:_)?|Ref)|ilter(?:Selector)?|ormat)|Iterator(?:_)?|Metrics|Notif(?:ication(?:InfoRef(?:_)?|Ref(?:_)?)|y(?:Action|Option))|Query(?:Callback|MessageID|SourceContext)|Ref|Size))|G(?:eneration|lyph(?:I(?:dealMetrics|nfoFlags)|Ref|ScreenMetrics|Vector))|Just(?:PriorityWidthDeltaOverrides|WidthDeltaEntryOverride)|L(?:ayoutRecord|ineLayoutOptions)|NotificationCallback|OptionFlags|Point|Quadratic(?:C(?:losePath(?:ProcPtr|UPP)|urve(?:ProcPtr|UPP))|Line(?:ProcPtr|UPP)|NewPath(?:ProcPtr|UPP))|StyleRenderingOptions|Trapezoid|U(?:Attribute(?:Info|Tag|ValuePtr)|Background(?:Color|Data(?:Type)?)|C(?:aret|ur(?:sorMovementType|vePath(?:s)?))|Direct(?:DataSelector|LayoutOperationOverride(?:ProcPtr|UPP))|F(?:latten(?:StyleRunOptions|edDataStreamFormat)|ont(?:F(?:allback(?:Method|s)|eature(?:Selector|Type))|ID|Variation(?:Axis|Value)))|Glyph(?:Info(?:Array)?|Selector)|HighlightMethod|L(?:ayoutOperation(?:CallbackStatus|OverrideSpecifier|Selector)|ine(?:Ref|Truncation))|RGBAlphaColor|Style(?:Comparison|LineCountType|RunInfo|SettingRef)?|T(?:ab(?:Type)?|ext(?:Layout|Measurement))|Un(?:FlattenStyleRunOptions|highlightData)|VerticalCharacterType))|U(?:3DMixer(?:AttenuationCurve|RenderingFlags)|ChannelInfo|D(?:ependentParameter|istanceAttenuationData)|EventListener(?:Block|Proc|Ref)|Graph|Host(?:Identifier|VersionIdentifier)|InputSamplesInOutputCallback(?:Struct)?|ListenerBase|MIDIOutputCallback(?:Struct)?|N(?:ode(?:Connection|Interaction|RenderCallback)?|umVersion)|P(?:arameter(?:EventType|Listener(?:Block|Proc|Ref)|MIDIMapping(?:Flags)?)|reset(?:Event)?)|Re(?:nderCallback(?:Struct)?|verbRoomType)|S(?:ampler(?:BankPresetData|InstrumentData)|cheduledAudioSliceFlags|patial(?:Mixer(?:AttenuationCurve|RenderingFlags)|izationAlgorithm)))|V(?:Audio(?:Integer|SessionErrorCode|UInteger)|L(?:CompareItems(?:ProcPtr|UPP)|DisposeItem(?:ProcPtr|UPP)|ItemSize(?:ProcPtr|UPP)|NodeType|Order|Tree(?:Ptr|Struct)|VisitStage|Walk(?:ProcPtr|UPP)))|X(?:CopyMultipleAttributeOptions|Error|MenuItemModifiers|Observer(?:Callback(?:WithInfo)?|Ref)|Priority|U(?:IElementRef|nderlineStyle)|Value(?:Ref|Type))|l(?:ert(?:Std(?:AlertParam(?:Ptr|Rec)|CFStringAlertParam(?:Ptr|Rec))|T(?:Hndl|Ptr|emplate|ype))|ias(?:Handle|InfoType|Ptr|Record))|n(?:chorPoint(?:Table)?|krTable)|pp(?:Parameters(?:Ptr)?|earance(?:PartCode|RegionCode)|l(?:eEvent(?:Ptr)?|icationSpecificChunk(?:Ptr)?))|reaID|sscEntry|u(?:dio(?:B(?:alanceFade(?:Type)?|uffer(?:List)?|ytePacketTranslation(?:Flags)?)|C(?:hannel(?:Bitmap|CoordinateIndex|Description|Flags|La(?:bel|yout(?:Tag)?))|lass(?:Description|ID)|o(?:dec(?:AppendInput(?:BufferListProc|DataProc)|GetProperty(?:InfoProc|Proc)|InitializeProc|MagicCookieInfo|Pr(?:imeInfo|o(?:duceOutput(?:BufferListProc|PacketsProc)|pertyID))|ResetProc|SetPropertyProc|UninitializeProc)?|mponent(?:Description|F(?:actoryFunction|lags)|Instan(?:ce|tiationOptions)|Method|PlugInInterface|ValidationResult)?|nverter(?:ComplexInputDataProc|InputDataProc|Pr(?:imeInfo|opertyID)|Ref)))|Device(?:I(?:D|O(?:Block|Proc(?:ID)?))|Property(?:ID|ListenerProc))|F(?:ile(?:Component(?:C(?:loseProc|ountUserDataProc|reateURLProc)|ExtensionIsThisFormatProc|FileDataIsThisFormatProc|Get(?:GlobalInfo(?:Proc|SizeProc)|Property(?:InfoProc|Proc)|UserData(?:Proc|SizeProc))|InitializeWithCallbacksProc|Op(?:en(?:URLProc|WithCallbacksProc)|timizeProc)|PropertyID|Re(?:ad(?:BytesProc|Packet(?:DataProc|sProc))|moveUserDataProc)|Set(?:PropertyProc|UserDataProc)|Write(?:BytesProc|PacketsProc))?|F(?:DFTable(?:Extended)?|lags)|ID|Marker(?:List)?|P(?:acketTableInfo|ermissions|ropertyID)|Region(?:Flags|List)?|Stream(?:ID|P(?:arseFlags|roperty(?:Flags|ID))|SeekFlags|_P(?:acketsProc|ropertyListenerProc))|Type(?:AndFormatID|ID)|_(?:GetSizeProc|ReadProc|S(?:MPTE_Time|etSizeProc)|WriteProc))|ormat(?:Flags|I(?:D|nfo)|ListItem|PropertyID)|ramePacketTranslation)|Hardware(?:IOProcStreamUsage|P(?:owerHint|roperty(?:ID|ListenerProc)))|IndependentPacketTranslation|LevelControlTransferFunction|O(?:bject(?:ID|Property(?:Address|Element|Listener(?:Block|Proc)|S(?:cope|elector)))|utputUnitSt(?:art(?:AtTimeParams|Proc)|opProc))|Pa(?:cket(?:DependencyInfoTranslation|R(?:angeByteCountTranslation|ollDistanceTranslation))|nning(?:Info|Mode))|Queue(?:Buffer(?:Ref)?|ChannelAssignment|InputCallback(?:Block)?|LevelMeterState|OutputCallback(?:Block)?|P(?:arameter(?:Event|ID|Value)|ro(?:cessingTap(?:Callback|Flags|Ref)|perty(?:ID|ListenerProc)))|Ref|TimelineRef)|RecordingChunk(?:Ptr)?|S(?:ampleType|e(?:rvices(?:PropertyID|SystemSoundCompletionProc)|ttingsFlags)|tream(?:BasicDescription|ID|P(?:acketDescription|ropertyListenerProc)|RangedDescription))|TimeStamp(?:Flags)?|Unit(?:Add(?:PropertyListenerProc|RenderNotifyProc)|Co(?:coaViewInfo|mplexRenderProc|nnection)|E(?:lement|vent(?:Type)?|xternalBuffer)|FrequencyResponseBin|GetP(?:arameterProc|roperty(?:InfoProc|Proc))|InitializeProc|M(?:IDIControlMapping|eterClipping)|NodeConnection|OtherPluginDesc|P(?:arameter(?:Event|HistoryInfo|I(?:D(?:Name)?|nfo)|NameInfo|Options|StringFromValue|Unit|Value(?:FromString|Name|Translation)?)?|r(?:esetMAS_Setting(?:Data|s)|o(?:cess(?:MultipleProc|Proc)|perty(?:ID|ListenerProc)?)))|Re(?:move(?:PropertyListener(?:Proc|WithUserDataProc)|RenderNotifyProc)|nder(?:ActionFlags|Proc)|setProc)|S(?:ampleType|c(?:heduleParametersProc|ope)|etP(?:arameterProc|ropertyProc))|UninitializeProc)?|Value(?:Range|Translation))|thorization(?:AsyncCallback|E(?:nvironment|xternalForm)|Flags|Item(?:Set)?|OpaqueRef|R(?:ef|ights)|String)))|B(?:T(?:HeaderRec|NodeDescriptor|reeKey(?:Limits)?)|asicWindowDescription|i(?:gEndian(?:Fixed|Long|OSType|Short|U(?:Int32|nsigned(?:Fixed|Long|Short)))|tMap(?:Handle|Ptr)?)|sln(?:Baseline(?:Class|Record)|Format(?:0Part|1Part|2Part|3Part|Union)|Table(?:Format|Ptr)?))|C(?:A(?:BarBeatTime|Clock(?:Beats|ListenerProc|Message|PropertyID|Ref|S(?:MPTEFormat|amples|econds|yncMode)|T(?:empo|ime(?:Format|base)?))|F(?:Audio(?:Description|FormatListItem)|ChunkHeader|DataChunk|F(?:ileHeader|ormatFlags)|In(?:foStrings|strumentChunk)|Marker(?:Chunk)?|Overview(?:Chunk|Sample)|P(?:acketTableHeader|eakChunk|ositionPeak)|Region(?:Chunk|Flags)?|String(?:ID|s)|UMIDChunk|_(?:SMPTE_Time|UUID_ChunkHeader))|MeterTrackEntry|T(?:empoMapEntry|ransform3D))|C(?:Tab(?:Handle|Ptr)|_(?:LONG(?:64)?|MD(?:2(?:_CTX|state_st)|4(?:_CTX|state_st)|5(?:_CTX|state_st))|SHA(?:1(?:_CTX|state_st)|256(?:_CTX|state_st)|512(?:_CTX|state_st))))|E_(?:CrlNumber|D(?:ataType|eltaCrl)|ExtendedKeyUsage|GeneralNameType)|F(?:H(?:TTP(?:AuthenticationRef|MessageRef)|ost(?:ClientC(?:allBack|ontext)|InfoType|Ref))|Net(?:Diagnostic(?:Ref|StatusValues)|Service(?:Browser(?:ClientCallBack|Flags|Ref)|ClientC(?:allBack|ontext)|Monitor(?:ClientCallBack|Ref|Type)|Re(?:f|gisterFlags)|sError)|workErrors)|ProxyAutoConfigurationResultCallback|StreamErrorHTTP(?:Authentication)?)|G(?:Image(?:AnimationStatus|Destination(?:Ref)?|Metadata(?:Errors|Ref|T(?:ag(?:Ref)?|ype))?|PropertyOrientation|Source(?:AnimationBlock|Ref|Status)?)|L(?:C(?:PContextPriorityRequest|ontext(?:Enable|Obj|Parameter))|Error|G(?:PURestartStatus|lobalOption)|OpenGLProfile|P(?:BufferObj|ixelFormat(?:Attribute|Obj))|Renderer(?:InfoObj|Property)|ShareGroup(?:Obj|Rec))|MutableImageMetadataRef|rafP(?:ort|tr))|M(?:2(?:Header|Profile(?:Ptr)?)|4Header|AdaptationMatrixType|B(?:itmap|ufferLocation)|C(?:MY(?:Color|KColor)|oncatProfileSet|urveType)|D(?:at(?:aType|eTime(?:Type)?)|evice(?:Class|Info|Profile(?:Array|Info)|Scope))|F(?:ixedXY(?:Color|ZColor)|l(?:atten(?:ProcPtr|UPP)|oatBitmap(?:Flags)?))|GrayColor|H(?:LSColor|SVColor|andleLocation)|IntentCRDVMSize|L(?:abColor|u(?:t(?:16Type|8Type)|vColor))|M(?:CreateTransformPropertyProc|Info|akeAndModel(?:Type)?|easurementType|ulti(?:Funct(?:CLUTType|Lut(?:A2BType|Type))|LocalizedUniCode(?:EntryRec|Type)|channel(?:5Color|6Color|7Color|8Color)))|Na(?:medColor(?:2(?:EntryType|Type)|Type)?|tiveDisplayInfo(?:Type)?)|P(?:S2CRDVMSizeType|a(?:rametricCurveType|thLocation)|rofile(?:IterateData|Location|SequenceDescType))|RGBColor|S(?:15Fixed16ArrayType|CertificateChainMode|DecoderRef|EncoderRef|Signe(?:dAttributes|rStatus)|creening(?:ChannelRec|Type)|ignatureType)|T(?:ag(?:ElemTable|Record)|ext(?:DescriptionType|Type))|U(?:16Fixed16ArrayType|Int(?:16ArrayType|32ArrayType|64ArrayType|8ArrayType)|crBgType|nicodeTextType)|Vi(?:deoCardGamma(?:Formula|T(?:able|ype))?|ewingConditionsType)|XYZ(?:Co(?:lor|mponent)|Type)|YxyColor)|QDProcs(?:Ptr)?|S(?:ComponentsThreadMode|DiskSpaceRecovery(?:Callback|Options)|Identity(?:AuthorityRef|Cl(?:ass|ientContext)|Flags|Query(?:ClientContext|Event|Flags|Re(?:ceiveEventCallback|f)|StringComparisonMethod)|Ref|StatusUpdatedCallback)|SM_(?:A(?:C(?:L_(?:AUTHORIZATION_TAG|EDIT_MODE|HANDLE|KEYCHAIN_PROMPT_SELECTOR|PR(?:EAUTH_TRACKING_STATE|OCESS_SUBJECT_SELECTOR)|SUBJECT_TYPE)|_HANDLE)|LGORITHMS|PPLE(?:CSPDL_DB_(?:CHANGE_PASSWORD_PARAMETERS(?:_PTR)?|IS_LOCKED_PARAMETERS(?:_PTR)?|SETTINGS_PARAMETERS(?:_PTR)?)|DL_OPEN_PARAMETERS(?:_PTR)?|_(?:CL_CSR_REQUEST|TP_(?:ACTION_(?:DATA|FLAGS)|C(?:ERT_REQUEST|RL_OPT(?:IONS|_FLAGS))|NAME_OID|S(?:MIME_OPTIONS|SL_OPTIONS))))|TT(?:ACH_FLAGS|RIBUTE_TYPE))|B(?:ER_TAG|ITMASK|OOL)|C(?:ALLOC|C_HANDLE|ERT(?:GROUP_TYPE(?:_PTR)?|_(?:BUNDLE_(?:ENCODING|TYPE)|ENCODING(?:_PTR)?|PARSE_FORMAT(?:_PTR)?|TYPE(?:_PTR)?))|L_(?:HANDLE|TEMPLATE_TYPE)|ONTEXT_(?:EVENT|TYPE)|RL(?:GROUP_TYPE(?:_PTR)?|_(?:ENCODING(?:_PTR)?|PARSE_FORMAT(?:_PTR)?|TYPE(?:_PTR)?))|SP(?:TYPE|_(?:FLAGS|HANDLE|READER_FLAGS)))|D(?:B_(?:A(?:CCESS_TYPE(?:_PTR)?|TTRIBUTE_(?:FORMAT(?:_PTR)?|NAME_FORMAT(?:_PTR)?))|CONJUNCTIVE(?:_PTR)?|HANDLE|INDEX(?:ED_DATA_LOCATION|_TYPE)|MODIFY_MODE|OPERATOR(?:_PTR)?|RE(?:CORDTYPE|TRIEVAL_MODES))|L(?:TYPE(?:_PTR)?|_(?:CUSTOM_ATTRIBUTES|FFS_ATTRIBUTES|HANDLE|LDAP_ATTRIBUTES|ODBC_ATTRIBUTES|PKCS11_ATTRIBUTE(?:_PTR)?)))|E(?:NCRYPT_MODE|VIDENCE_FORM)|FREE|H(?:ANDLE(?:_PTR)?|EADERVERSION)|INTPTR|K(?:EY(?:ATTR_FLAGS|BLOB_(?:FORMAT|TYPE)|CLASS|USE|_(?:HIERARCHY|TYPE))|R(?:SP_HANDLE|_POLICY_(?:FLAGS|TYPE)))|L(?:IST_(?:ELEMENT_(?:PTR|TYPE(?:_PTR)?)|TYPE(?:_PTR)?)|ONG_HANDLE(?:_PTR)?)|M(?:A(?:LLOC|NAGER_EVENT_TYPES)|ODULE_(?:EVENT(?:_PTR)?|HANDLE(?:_PTR)?))|NET_(?:ADDRESS_TYPE|PROTOCOL)|P(?:ADDING|KCS(?:5_PBKDF2_PRF|_OAEP_(?:MGF|PSOURCE))|R(?:IVILEGE(?:_SCOPE)?|OC_ADDR(?:_PTR)?)|VC_MODE)|QUERY_FLAGS|RE(?:ALLOC|TURN)|S(?:AMPLE_TYPE|C_FLAGS|ERVICE_(?:MASK|TYPE)|IZE|TRING)|T(?:IMESTRING|P_(?:A(?:CTION|PPLE_(?:CERT_STATUS|EVIDENCE_HEADER)|UTHORITY_REQUEST_TYPE(?:_PTR)?)|C(?:ERT(?:CHANGE_(?:ACTION|REASON|STATUS)|ISSUE_STATUS|NOTARIZE_STATUS|RECLAIM_STATUS|VERIFY_STATUS)|ONFIRM_STATUS(?:_PTR)?|RLISSUE_STATUS)|FORM_TYPE|HANDLE|S(?:ERVICES|TOP_ON)))|USEE_TAG|WORDID_TYPE|X509(?:EXT_DATA_FORMAT|_OPTION))|pecArray)|T(?:CharacterCollection|F(?:ont(?:Collection(?:CopyOptions|Ref|SortDescriptorsCallback)|Descriptor(?:MatchingState|Ref)|Format|Manager(?:AutoActivationSetting|Error|Scope)|O(?:ptions|rientation)|Priority|Ref|S(?:tylisticClass|ymbolicTraits)|Table(?:Options|Tag)|UIFontType)|rame(?:P(?:athFillRule|rogression)|Ref|setterRef))|GlyphInfoRef|Line(?:B(?:oundsOptions|reakMode)|Ref|TruncationType)|MutableFontCollectionRef|ParagraphStyle(?:Ref|S(?:etting|pecifier))|Ru(?:by(?:A(?:lignment|nnotationRef)|Overhang|Position)|n(?:Delegate(?:Callbacks|DeallocateCallback|Get(?:AscentCallback|DescentCallback|WidthCallback)|Ref)|Ref|Status))|T(?:ext(?:Alignment|TabRef)|ypesetterRef)|UnderlineStyle(?:Modifiers)?|WritingDirection|ab(?:Handle|Ptr))|V(?:AttachmentMode|BufferRef|DisplayLink(?:Output(?:Callback|Handler)|Ref)|FillExtendedPixelsCallBack(?:Data)?|ImageBufferRef|Op(?:enGL(?:Buffer(?:PoolRef|Ref)|Texture(?:CacheRef|Ref))|tionFlags)|P(?:ixelBuffer(?:LockFlags|Pool(?:FlushFlags|Ref)|Re(?:f|lease(?:BytesCallback|PlanarBytesCallback)))|lanar(?:ComponentInfo|PixelBufferInfo(?:_YCbCr(?:BiPlanar|Planar))?))|Return|SMPTETime(?:Flags|Type)?|Time(?:Flags|Stamp(?:Flags)?)?)|a(?:l(?:ibrat(?:e(?:Event(?:ProcPtr|UPP)|ProcPtr|UPP)|orInfo)|lingConventionType)|nCalibrate(?:ProcPtr|UPP)|retHook(?:ProcPtr|UPP)|tPositionRec)|ell|h(?:ar(?:ByteTable|s(?:Handle|Ptr)?)|unkHeader)|lickActivationResult|o(?:l(?:l(?:atorRef|ection(?:Exception(?:ProcPtr|UPP)|Flatten(?:ProcPtr|UPP)|Tag)?)|or(?:C(?:hangedUPP|omplement(?:ProcPtr|UPP))|S(?:earch(?:ProcPtr|UPP)|pec(?:Ptr)?|ync(?:AlphaInfo|CMM(?:Ref)?|Data(?:Depth|Layout)|M(?:D5|utableProfileRef)|Profile(?:Ref)?|Transform(?:Ref)?))|Table))|m(?:m(?:ent(?:Type|sChunk(?:Ptr)?)?|onChunk(?:Ptr)?)|ponent(?:AliasResource|Description|FunctionUPP|Instance(?:Record)?|MPWorkFunction(?:HeaderRecord(?:Ptr)?|ProcPtr|UPP)|P(?:arameters|latformInfo(?:Array)?)|R(?:e(?:cord|s(?:ource(?:Extension|Handle|Ptr)?|ult))|outine(?:ProcPtr|UPP)))?)|n(?:st(?:ATSUAttributeValuePtr|FS(?:EventStreamRef|SpecPtr)|HFSUniStr255Param|ScriptCodeRunPtr|Text(?:EncodingRunPtr|Ptr|ToUnicodeInfo)|Uni(?:CharArrayPtr|code(?:MappingPtr|ToTextInfo)))|t(?:ainerChunk|extualMenuInterfaceStruct|rol(?:Action(?:ProcPtr|UPP)|B(?:evel(?:Button(?:Behavior|Menu(?:Behavior|Placement))|Thickness)|utton(?:ContentInfo(?:Ptr)?|GraphicAlignment|Text(?:Alignment|Placement)))|C(?:lock(?:Flags|Type)|ontentType)|DisclosureTriangleOrientation|EditText(?:Selection(?:Ptr|Rec)|Validation(?:ProcPtr|UPP))|Fo(?:cusPart|ntStyle(?:Ptr|Rec))|Handle|I(?:D|mageContentInfo(?:Ptr)?)|K(?:ey(?:Filter(?:ProcPtr|Result|UPP)|ScriptBehavior)|ind)|P(?:artCode|opupArrow(?:Orientation|Size)|ushButtonIconAlignment)|R(?:ef|oundButtonSize)|S(?:ize|liderOrientation)|T(?:ab(?:Direction|Entry|InfoRec(?:V1)?|Size)|emplate(?:Handle|Ptr)?)|UserPane(?:Activate(?:ProcPtr|UPP)|Draw(?:ProcPtr|UPP)|Focus(?:ProcPtr|UPP)|HitTest(?:ProcPtr|UPP)|Idle(?:ProcPtr|UPP)|KeyDown(?:ProcPtr|UPP)|Tracking(?:ProcPtr|UPP))|Variant)))|reEndianFlipProc|untUserDataFDF)|tlCTab|ustomBadgeResource(?:Handle|Ptr)?)|D(?:A(?:ApprovalSessionRef|DiskRef|SessionRef)|C(?:M(?:AccessMethod(?:Feature|I(?:D|terator))|Dictionary(?:Header|I(?:D|terator)|Ref|StreamRef)|F(?:i(?:eld(?:Attributes|T(?:ag|ype))|ndMethod)|oundRecordIterator)|Object(?:I(?:D|terator)|Ref)|ProgressFilter(?:ProcPtr|UPP)|UniqueID)|SDictionaryRef)|ER(?:Byte|Item|Size)|I(?:TLMethod|nfo)|R(?:AudioTrackRef|BurnRef|CDTextBlockRef|DeviceRef|EraseRef|F(?:SObjectRef|ile(?:Fork(?:Index|Size(?:Info|Query))|Message|Pro(?:c|ductionInfo)|Ref|system(?:Mask|TrackRef))|olderRef)|LinkType|NotificationC(?:allback|enterRef)|RefCon(?:Callbacks|Re(?:leaseCallback|tainCallback))|T(?:rack(?:CallbackProc|Message|ProductionInfo|Ref)|ypeRef))|XInfo|at(?:a(?:Array|Browser(?:A(?:cce(?:ptDrag(?:ProcPtr|UPP)|ssibilityItemInfo(?:V(?:0|1))?)|ddDragItem(?:ProcPtr|UPP))|C(?:allbacks|ustomCallbacks)|Dra(?:gFlags|wItem(?:ProcPtr|UPP))|Edit(?:Command|Item(?:ProcPtr|UPP))|GetContextualMenu(?:ProcPtr|UPP)|HitTest(?:ProcPtr|UPP)|Item(?:AcceptDrag(?:ProcPtr|UPP)|Compare(?:ProcPtr|UPP)|D(?:ata(?:ProcPtr|Ref|UPP)|ragRgn(?:ProcPtr|UPP))|HelpContent(?:ProcPtr|UPP)|ID|Notification(?:ProcPtr|UPP|WithItem(?:ProcPtr|UPP))?|ProcPtr|ReceiveDrag(?:ProcPtr|UPP)|State|UPP)|ListView(?:ColumnDesc|HeaderDesc|PropertyFlags)|Metric|P(?:ostProcessDrag(?:ProcPtr|UPP)|roperty(?:Desc|Flags|ID|Part|Type))|Re(?:ceiveDrag(?:ProcPtr|UPP)|vealOptions)|S(?:e(?:lect(?:ContextualMenu(?:ProcPtr|UPP)|ion(?:AnchorDirection|Flags))|tOption)|ortOrder)|T(?:ableView(?:Column(?:Desc|I(?:D|ndex))|HiliteStyle|PropertyFlags|RowIndex)|racking(?:ProcPtr|Result|UPP))|ViewStyle)|Handle|Ptr)|e(?:Cache(?:Ptr|Record)|Delta|Form|Orders|TimeRec))|e(?:bug(?:AssertOutputHandler(?:ProcPtr|UPP)|ComponentCallback(?:ProcPtr|UPP)|ger(?:DisposeThread(?:ProcPtr|TPP|UPP)|NewThread(?:ProcPtr|TPP|UPP)|ThreadScheduler(?:ProcPtr|TPP|UPP)))|ferredTask(?:P(?:rocPtr|tr)|UPP)?|lim(?:Type|iterInfo)|s(?:cType|kHook(?:ProcPtr|UPP)))|ialog(?:Item(?:Index(?:ZeroBased)?|Type)|P(?:lacementSpec|tr)|Ref|T(?:Hndl|Ptr|emplate))|o(?:Get(?:FileTranslationListProcPtr|ScrapTranslationListProcPtr|TranslatedFilenameProcPtr)|Identify(?:FileProcPtr|ScrapProcPtr)|Translate(?:FileProcPtr|ScrapProcPtr)|cOpenMethod)|ra(?:g(?:A(?:ctions|ttributes)|Behaviors|Constraint|Drawing(?:ProcPtr|UPP)|GrayRgn(?:ProcPtr|UPP)|I(?:mageFlags|nput(?:ProcPtr|UPP)|temRef)|Re(?:ceiveHandler(?:ProcPtr|UPP)|f(?:erence)?|gionMessage)|SendData(?:ProcPtr|UPP)|Tracking(?:Handler(?:ProcPtr|UPP)|Message))|wHook(?:ProcPtr|UPP)))|E(?:OLHook(?:ProcPtr|UPP)|ditUnicodePostUpdate(?:ProcPtr|UPP)|v(?:Cmd|QEl(?:Ptr)?|ent(?:Attributes|C(?:lass(?:ID)?|omparator(?:ProcPtr|UPP))|H(?:andler(?:CallRef|ProcPtr|Ref|UPP)|otKey(?:ID|Ref))|Kind|Loop(?:IdleTimer(?:Message|ProcPtr|UPP)|Ref|Timer(?:ProcPtr|Ref|UPP))|M(?:ask|o(?:difiers|use(?:Button|WheelAxis)))|P(?:aram(?:Name|Type)|riority)|QueueRef|Re(?:cord|f)|T(?:argetRef|ime(?:out|rInterval)?|ype(?:Spec)?)))|x(?:ception(?:Handler(?:ProcPtr|TPP|UPP)?|Info(?:rmation(?:PowerPC)?)?|Kind)|t(?:AudioFile(?:PropertyID|Ref)|Com(?:monChunk(?:Ptr)?|ponentResource(?:Handle|Ptr)?)|ended(?:AudioFormatInfo|ControlEvent|F(?:ileInfo|olderInfo)|NoteOnEvent|TempoEvent))))|F(?:CFontDescriptorRef|I(?:LE|nfo)|KEY(?:ProcPtr|UPP)|M(?:F(?:ilter(?:Selector)?|ont(?:CallbackFilter(?:ProcPtr|UPP)|DirectoryFilter|Family(?:CallbackFilter(?:ProcPtr|UPP)|I(?:nstance(?:Iterator)?|terator))?|Iterator|S(?:ize|tyle))?)|Generation|Input)|N(?:Message|Subscription(?:ProcPtr|Ref|UPP))|P(?:RegIntel|UInformation(?:Intel64|PowerPC)?)|S(?:Al(?:ias(?:FilterProcPtr|Info(?:Bitmap|Ptr)?)|locationFlags)|Catalog(?:BulkParam(?:Ptr)?|Info(?:Bitmap|Ptr)?)|E(?:jectStatus|ventStream(?:C(?:allback|ontext|reateFlags)|Event(?:Flags|Id)|Ref))|F(?:ile(?:Operation(?:ClientContext|Ref|Sta(?:ge|tusProcPtr))|SecurityRef)|ork(?:CBInfoParam(?:Ptr)?|I(?:OParam(?:Ptr)?|nfo(?:Flags|Ptr)?)))|I(?:ORefNum|terator(?:Flags)?)|MountStatus|P(?:athFileOperationStatusProcPtr|ermissionInfo)|R(?:angeLockParam(?:Ptr)?|ef(?:ForkIOParam(?:Ptr)?|P(?:aram(?:Ptr)?|tr))?)|S(?:earchParams(?:Ptr)?|pec(?:ArrayPtr|Handle|Ptr)?)|UnmountStatus|Volume(?:Eject(?:ProcPtr|UPP)|Info(?:Bitmap|P(?:aram(?:Ptr)?|tr))?|Mount(?:ProcPtr|UPP)|Operation|RefNum|Unmount(?:ProcPtr|UPP)))|Vector|XInfo|amRec|ile(?:Info|T(?:ranslation(?:List(?:Handle|Ptr)?|Spec(?:Array(?:Handle|Ptr))?)|ype(?:Spec)?))|lavor(?:Flags|Type)|ndr(?:DirInfo|Extended(?:DirInfo|FileInfo)|FileInfo|OpaqueInfo)|o(?:lder(?:Class|Desc(?:Flags|Ptr)?|Info|Location|ManagerNotification(?:ProcPtr|UPP)|Routing(?:Ptr)?|Type)|nt(?:Assoc|Info|LanguageCode|NameCode|PlatformCode|Rec(?:Hdl|Ptr)?|S(?:criptCode|electionQDStyle(?:Ptr)?)|Variation)|rmat(?:Class|ResultType|Status|VersionChunk(?:Ptr)?)))|G(?:D(?:Handle|Ptr|evice)|L(?:b(?:itfield|oolean|yte)|c(?:har(?:ARB)?|lamp(?:d|f))|double|enum|f(?:ixed|loat)|ha(?:lf(?:ARB)?|ndleARB)|int(?:64(?:EXT)?|ptr(?:ARB)?)?|s(?:hort|izei(?:ptr(?:ARB)?)?|ync)|u(?:byte|int(?:64(?:EXT)?)?|short)|void)|NEFilterUPP|World(?:Flags|Ptr)|e(?:nericID|t(?:GrowImageRegionRec|MissingComponentResource(?:ProcPtr|UPP)|NextEventFilter(?:ProcPtr|UPP)|Property(?:FDF|InfoFDF)|ScrapData(?:ProcPtr|UPP)?|UserData(?:FDF|SizeFDF)|VolParmsInfoBuffer|WindowRegion(?:Ptr|Rec(?:Ptr)?)))|lyph(?:Collection|ID)|raf(?:P(?:ort|tr)|Verb))|H(?:FS(?:Catalog(?:F(?:ile|older)|Key|NodeID|Thread)|Extent(?:Descriptor|Key|Record)|Flavor|MasterDirectoryBlock|Plus(?:Attr(?:Data|Extents|ForkData|InlineData|Key|Record)|BSDInfo|Catalog(?:F(?:ile|older)|Key|Thread)|Extent(?:Descriptor|Key|Record)|ForkData|VolumeHeader)|UniStr255)|I(?:A(?:rchiveRef|xis(?:Position|Scale))|Binding(?:Kind)?|Co(?:mmand(?:Extended)?|ntentBorderMetrics|ordinateSpace)|DelegatePosition|ImageViewAutoTransformOptions|LayoutInfo|M(?:odalClickResult|utableShapeRef)|Object(?:ClassRef|Ref)|Po(?:int|sition(?:Kind|ing))|Rect|S(?:c(?:al(?:eKind|ing)|roll(?:BarTrackInfo|ViewAction))|egmentBehavior|hape(?:EnumerateProcPtr|Ref)|i(?:deBinding|ze))|T(?:heme(?:Animation(?:FrameInfo|TimeInfo)|B(?:ackgroundDrawInfo(?:Ptr)?|uttonDrawInfo(?:Ptr)?)|ChasingArrowsDrawInfo(?:Ptr)?|F(?:ocusRing|rame(?:DrawInfo(?:Ptr)?|Kind))|Gr(?:abberDrawInfo(?:Ptr)?|o(?:upBox(?:DrawInfo(?:Ptr)?|Kind)|wBox(?:DrawInfo(?:Ptr)?|Kind|Size)))|Header(?:DrawInfo(?:Ptr)?|Kind)|Menu(?:BarDrawInfo(?:Ptr)?|DrawInfo(?:Ptr|VersionZero(?:Ptr)?)?|ItemDrawInfo(?:Ptr)?|TitleDrawInfo(?:Ptr)?)|Orientation|P(?:lacardDrawInfo(?:Ptr)?|opupArrowDrawInfo(?:Ptr)?)|S(?:crollBarDelimitersDrawInfo(?:Ptr)?|e(?:gment(?:Adornment|DrawInfo(?:Ptr)?|Kind|Position|Size)|paratorDrawInfo(?:Ptr)?)|plitter(?:Adornment|DrawInfo(?:Ptr)?))|T(?:ab(?:Adornment|DrawInfo(?:VersionZero)?|Kind|P(?:ane(?:Adornment|DrawInfo(?:VersionZero)?)|osition)|Size)|ext(?:BoxOptions|HorizontalFlush|Info|Truncation|VerticalFlush)|ickMarkDrawInfo(?:Ptr)?|rackDrawInfo)|Window(?:DrawInfo(?:Ptr)?|WidgetDrawInfo(?:Ptr)?))|oolbar(?:Display(?:Mode|Size)|ItemRef|Ref)|ypeAndCreator)|View(?:Content(?:Info(?:Ptr)?|Type)|F(?:eatures|rameMetrics)|I(?:D|mageContent(?:Info|Type))|Kind|PartCode|Ref|TrackingArea(?:ID|Ref)|ZOrderOp)|Window(?:Availability|BackingLocation|Depth|Ref|S(?:caleMode|haringType)))|M(?:Cont(?:ent(?:ProvidedType|Request|Type)|rolContent(?:ProcPtr|UPP))|HelpContent(?:Ptr|Rec)?|Menu(?:ItemContent(?:ProcPtr|UPP)|TitleContent(?:ProcPtr|UPP))|StringResType|TagDisplaySide|WindowContent(?:ProcPtr|UPP)|enuBar(?:Header|Menu))|i(?:ghHook(?:ProcPtr|UPP)|liteMenuItemData(?:Ptr)?|tTestHook(?:ProcPtr|UPP))|o(?:mograph(?:Accent|DicInfoRec|Weight)|stCallback(?:Info|_Get(?:BeatAndTempo|MusicalTimeLocation|TransportState(?:2)?))))|I(?:BNibRef|C(?:A(?:ppSpec(?:Handle|List(?:Handle|Ptr)?|Ptr)?|ttr)|CharTable(?:Handle|Ptr)?|F(?:i(?:leSpec(?:Handle|Ptr)?|xedLength)|ontRecord(?:Handle|Ptr)?)|Instance|MapEntry(?:Flags|Handle|Ptr)?|P(?:erm|rofileID(?:Ptr)?)|Service(?:Entry(?:Flags|Handle|Ptr)?|s(?:Handle|Ptr)?))|O(?:A(?:ddressRange|lignment|ppleTimingID|syncC(?:allback(?:0|1|2)?|ompletionContent))|ByteCount(?:32|64)?|C(?:acheMode|o(?:lor(?:Component|Entry)|mpletion(?:ProcPtr|UPP)))|D(?:e(?:tailedTimingInformation(?:V(?:1|2))?|viceNumber)|isplay(?:ModeI(?:D|nformation)|ProductID|ScalerInformation|TimingRange(?:V(?:1|2))?|VendorID))|F(?:B(?:D(?:PLinkConfig|isplayModeDescription)|HDRMetaData(?:V1)?)|ixed(?:1616|Point32)?|ramebufferInformation)|G(?:Bounds|Point|Size)|HardwareCursor(?:Descriptor|Info)|I(?:ndex|temCount)|LogicalAddress|N(?:amedValue|otificationPort(?:Ref)?)|OptionBits|P(?:hysical(?:Address(?:32|64)?|Length(?:32|64)?|Range)|ixel(?:Aperture|Encoding|Information))|Return|S(?:e(?:lect|rvice(?:InterestC(?:allback|ontent(?:64)?)|MatchingCallback))|urface(?:Component(?:Name|Range|Type)|ID|LockOptions|PurgeabilityState|Ref|Subsampling))|TimingInformation|V(?:ersion|irtual(?:Address|Range)))|SAType|con(?:A(?:ction(?:ProcPtr|UPP)|lignmentType)|Family(?:Element|Handle|Ptr|Resource)|Getter(?:ProcPtr|UPP)|Ref|Se(?:lectorValue|rvicesUsageFlags)|TransformType)|n(?:d(?:exToUCString(?:ProcPtr|UPP)|icatorDragConstraint(?:Ptr)?)|strumentChunk(?:Ptr)?|t(?:erfaceTypeList|l(?:0(?:Hndl|Ptr|Rec)|1(?:Hndl|Ptr|Rec)|Text)))|t(?:emReference|l(?:1ExtRec|4(?:Handle|Ptr|Rec)|5Record|b(?:ExtRecord|Record)|cRecord)))|J(?:S(?:C(?:har|lass(?:Attributes|Definition|Ref)|ontext(?:GroupRef|Ref))|GlobalContextRef|Object(?:C(?:allAs(?:ConstructorCallback|FunctionCallback)|onvertToTypeCallback)|FinalizeCallback|GetProperty(?:Callback|NamesCallback)|InitializeCallback|Ref)|Property(?:Attributes|NameA(?:ccumulatorRef|rrayRef))|St(?:atic(?:Function|Value)|ringRef)|Type(?:dArrayBytesDeallocator)?|ValueRef)|apanesePartOfSpeech|ournalInfoBlock|ust(?:DirectionTable|P(?:C(?:Action(?:Subrecord|Type)?|ConditionalAddAction|D(?:ecompositionAction|uctilityAction)|GlyphRepeatAddAction|UnconditionalAddAction)|ostcompTable)|Table|WidthDelta(?:Entry|Group)|ificationFlags))|K(?:C(?:A(?:ttr(?:Type|ibute(?:List)?)|uthType)|C(?:allback(?:Info|ProcPtr|UPP)|ert(?:AddOptions|SearchOptions))|Event(?:Mask)?|Item(?:Attr|Class|Ref)|P(?:rotocolType|ublicKeyHash)|Ref|S(?:earchRef|tatus)|VerifyStopOn)|e(?:r(?:n(?:ArrayOffset|Entry|FormatSpecificHeader|IndexArrayHeader|Kerning(?:Pair|Value)|O(?:ffsetTable(?:Ptr)?|rderedList(?:Entry(?:Ptr)?|Header))|Pair|S(?:impleArrayHeader|tate(?:Entry|Header)|ubtable(?:Header(?:Ptr)?|Info))|Table(?:Format|Header(?:Handle|Ptr)?)?|Version0(?:Header|SubtableHeader))|x(?:A(?:nchorPointAction|rrayOffset)|Co(?:ntrolPoint(?:Action|Entry|Header)|ordinateAction)|FormatSpecificHeader|IndexArrayHeader|KerningPair|OrderedList(?:Entry(?:Ptr)?|Header)|S(?:impleArrayHeader|tate(?:Entry|Header)|ubtable(?:Coverage|Header(?:Ptr)?))|TableHeader(?:Handle|Ptr)?))|y(?:Map(?:ByteArray)?|boardLayout(?:Identifier|Kind|PropertyTag|Ref))))|L(?:A(?:ContextRef|EnvironmentRef|Homograph|Morpheme(?:Bundle|Path|Rec|sArray(?:Ptr)?)?|Property(?:Key|Type))|H(?:Element|Handle|Ptr|Table)|LCStyleInfo|S(?:A(?:cceptanceFlags|pplicationParameters)|HandlerOptions|ItemInfo(?:Flags|Record)|Launch(?:F(?:SRefSpec|lags)|URLSpec)|R(?:equestedInfo|olesMask)|SharedFileList(?:ChangedProcPtr|ItemRef|Re(?:f|solutionFlags)))|aunch(?:Flags|P(?:BPtr|aramBlockRec))|carCaret(?:ClassEntry|Table(?:Ptr)?)|ist(?:Bounds|ClickLoop(?:ProcPtr|UPP)|Def(?:ProcPtr|Spec(?:Ptr)?|Type|UPP)|Handle|Ptr|Re(?:c|f)|Search(?:ProcPtr|UPP))|o(?:cal(?:DateTime(?:Handle|Ptr)?|e(?:AndVariant|NameMask|Operation(?:Class|Variant)|PartMask|Ref))|ngDate(?:Cvt|Field|Rec|Time))|tag(?:StringRange|Table))|M(?:BarHook(?:ProcPtr|UPP)|C(?:Entry(?:Ptr)?|Table(?:Handle|Ptr)?)|D(?:EF(?:Draw(?:Data(?:Ptr)?|ItemsData(?:Ptr)?)|FindItemData(?:Ptr)?|HiliteItemData(?:Ptr)?)|ItemRef|Label(?:Domain|Ref)|Query(?:BatchingParams|Create(?:ResultFunction|ValueFunction)|OptionFlags|Ref|Sort(?:ComparatorFunction|OptionFlags))|S_HANDLE)|IDI(?:C(?:hannelMessage|lientRef|ompletionProc)|D(?:ataChunk(?:Ptr)?|eviceRef)|En(?:dpointRef|tityRef)|IOErrorNotification|MetaEvent|Not(?:eMessage|if(?:ication(?:MessageID)?|y(?:Block|Proc)))|Object(?:AddRemoveNotification|PropertyChangeNotification|Ref|Type)|P(?:acket(?:List)?|ortRef)|R(?:awData|ead(?:Block|Proc))|SysexSendRequest|TimeStamp|UniqueID)|P(?:A(?:ddressSpaceI(?:D|nfo)|reaID)|C(?:o(?:herenceID|nsoleID)|puID|riticalRegionI(?:D|nfo))|DebuggerLevel|E(?:G4ObjectID|vent(?:Flags|I(?:D|nfo))|xceptionKind)|IsFullyInitializedProc|NotificationI(?:D|nfo)|OpaqueID(?:Class)?|P(?:ageSizeClass|rocessID)|QueueI(?:D|nfo)|Remote(?:Context|Procedure)|Semaphore(?:Count|I(?:D|nfo))|T(?:ask(?:I(?:D|nfo(?:Version2)?)|Options|StateKind|Weight)|imerID))|a(?:c(?:Polygon|hine(?:Information(?:Intel64|PowerPC)?|Location))|gicCookieInfo|rker(?:Chunk(?:Ptr)?|IdType)?)|e(?:asureWindowTitleRec(?:Ptr)?|mory(?:ExceptionInformation|ReferenceKind)|nu(?:Attributes|Bar(?:Def(?:ProcPtr|UPP)|H(?:andle|eader)|Menu)|C(?:Rsrc(?:Handle|Ptr)?|ommand)|Def(?:Spec(?:Ptr)?|Type|UPP)|EventOptions|H(?:andle|ook(?:ProcPtr|UPP))|I(?:D|tem(?:Attributes|D(?:ata(?:Flags|Ptr|Rec)|rawing(?:ProcPtr|UPP))|I(?:D|ndex)))|Ref|T(?:itleDrawing(?:ProcPtr|UPP)|racking(?:Data(?:Ptr)?|Mode))))|ixe(?:dModeStateRecord|rDistanceParams)|o(?:dalFilter(?:ProcPtr|UPP|YD(?:ProcPtr|UPP))|r(?:pheme(?:PartOfSpeech|TextRange)|t(?:C(?:hain|ontextualSubtable)|FeatureEntry|InsertionSubtable|Ligature(?:ActionEntry|Subtable)|RearrangementSubtable|S(?:pecificSubtable|ubtable(?:MaskFlags)?|washSubtable)|Table)|x(?:C(?:hain|ontextualSubtable)|InsertionSubtable|LigatureSubtable|RearrangementSubtable|S(?:pecificSubtable|ubtable)|Table))|useTrackingResult)|usic(?:Device(?:Component|GroupID|InstrumentID|MIDIEventProc|NoteParams|S(?:t(?:artNoteProc|dNoteParams|opNoteProc)|ysExProc))|Event(?:Iterator|Type|UserData)|Player|Sequence(?:File(?:Flags|TypeID)|LoadFlags|Type|UserCallback)?|T(?:imeStamp|rack(?:LoopInfo)?)))|N(?:C(?:M(?:ConcatProfileS(?:et|pec)|DeviceProfileInfo)|olor(?:Changed(?:ProcPtr|UPP)|PickerInfo))|DR_record_t|Itl4(?:Handle|Ptr|Rec)|M(?:ProcPtr|Rec(?:Ptr)?|UPP)|PMColor(?:Ptr)?|WidthHook(?:ProcPtr|UPP)|X(?:ByteOrder|Coord|Event(?:Data|Ext(?:ension)?|Ptr|System(?:Device(?:List)?|Info(?:Data|Type)))?|KeyMapping|Mouse(?:Button|Scaling)|Point|S(?:ize|wapped(?:Double|Float))|TabletP(?:ointData(?:Ptr)?|roximityData(?:Ptr)?))|a(?:meTable|noseconds)|ote(?:InstanceID|ParamsControlValue)|u(?:llSt(?:Handle|Ptr|Rec)|m(?:FormatString(?:Rec)?|berParts(?:Ptr)?)))|O(?:S(?:A(?:Active(?:ProcPtr|UPP)|CreateAppleEvent(?:ProcPtr|UPP)|Error|ID|Send(?:ProcPtr|UPP)|syncReference(?:64)?|tomic_int64_aligned64_t)|FifoQueueHead|L(?:A(?:ccessor(?:ProcPtr|UPP)|djustMarks(?:ProcPtr|UPP))|Co(?:mpare(?:ProcPtr|UPP)|unt(?:ProcPtr|UPP))|DisposeToken(?:ProcPtr|UPP)|Get(?:ErrDesc(?:ProcPtr|UPP)|MarkToken(?:ProcPtr|UPP))|Mark(?:ProcPtr|UPP))|NotificationHeader(?:64)?|QueueHead)|ff(?:Pair|set(?:Array(?:Handle|Ptr)?|Table))|p(?:aque(?:A(?:E(?:DataStorageType|StreamRef)|TSU(?:FontFallbacks|Style|TextLayout)|UGraph|reaID|udio(?:Co(?:mponent|nverter)|FileStreamID|Queue(?:ProcessingTap|Timeline)?))|C(?:AClock|M(?:ProfileRef|WorldRef)|o(?:ll(?:atorRef|ection)|ntrolRef))|D(?:CM(?:FoundRecordIterator|Object(?:I(?:D|terator)|Ref))|ialogPtr|ragRef)|E(?:vent(?:H(?:andler(?:CallRef|Ref)|otKeyRef)|LoopRef|QueueRef|Ref|TargetRef)|xtAudioFile)|F(?:CFontDescriptorRef|NSubscriptionRef|S(?:Iterator|VolumeOperation))|GrafPtr|HI(?:ArchiveRef|Object(?:ClassRef|Ref)|ViewTrackingAreaRef)|I(?:BNibRef|CInstance|conRef)|JS(?:C(?:lass|ontext(?:Group)?)|PropertyNameA(?:ccumulator|rray)|String|Value)|KeyboardLayoutRef|L(?:A(?:ContextRef|EnvironmentRef)|SSharedFileList(?:ItemRef|Ref)|ocaleRef)|M(?:P(?:A(?:ddressSpaceID|reaID)|C(?:o(?:herenceID|nsoleID)|puID|riticalRegionID)|EventID|NotificationID|OpaqueID|ProcessID|QueueID|SemaphoreID|T(?:askID|imerID))|enuRef|usic(?:EventIterator|Player|Sequence|Track))|P(?:M(?:P(?:a(?:geFormat|per)|r(?:eset|int(?:Se(?:ssion|ttings)|er)))|Server)|asteboardRef|icker|olicySearchRef)|RgnHandle|S(?:RSpeechObject|crapRef|ec(?:AccessRef|CertificateRef|Identity(?:Ref|SearchRef)|KeyRef|TransformImplementation))|T(?:EC(?:ObjectRef|SnifferObjectRef)|SMDocumentID|XNObject|ext(?:BreakLocatorRef|ToUnicodeInfo)|hemeDrawingState|oolboxObjectClassRef|ranslationRef)|U(?:CTypeSelectRef|RLReference|nicodeToText(?:Info|RunInfo))|W(?:S(?:MethodInvocationRef|ProtocolHandlerRef)|indow(?:GroupRef|Ptr)))|bd(?:SideValues|Table(?:Format)?)|enCPicParams))|P(?:EF(?:ContainerHeader|ExportedSymbol(?:HashSlot|Key)?|Imported(?:Library|Symbol)|Loader(?:InfoHeader|RelocationHeader)|RelocChunk|S(?:ectionHeader|plitHashWord))|M(?:BorderType|ColorSpaceModel|D(?:ataFormat|estinationType|uplexMode)|La(?:nguageInfo|youtDirection)|O(?:bject|rientation)|P(?:PDDomain|a(?:ge(?:Format|ToPaperMappingType)|per(?:Margins|Type)?)|r(?:eset|int(?:DialogOptionFlags|Se(?:ssion|ttings)|er(?:State)?)))|QualityMode|Re(?:ct|solution)|S(?:calingAlignment|erver))|a(?:r(?:am(?:BlockRec|eterEvent)|mBlkPtr)|steboard(?:FlavorFlags|ItemID|PromiseKeeperProcPtr|Ref|S(?:tandardLocation|yncFlags))|t(?:Handle|Ptr|tern))|h(?:oneme(?:Descriptor|Info)|ysicalKeyboardLayoutType)|i(?:c(?:Handle|Ptr|ker(?:MenuItemInfo)?|ture)|x(?:Map(?:Handle|Ptr)?|Pat(?:Handle|Ptr)?))|lotIconRefFlags|oly(?:Handle|Ptr|gon)|r(?:interStatusOpcode|o(?:c(?:InfoType|ess(?:ApplicationTransformState|Info(?:ExtendedRec(?:Ptr)?|Rec(?:Ptr)?)))|gressTrackInfo|miseHFSFlavor|p(?:CharProperties|LookupS(?:egment|ingle)|Table|erty(?:Creator|Tag)))))|Q(?:D(?:Arc(?:ProcPtr|UPP)|Bits(?:ProcPtr|UPP)|Comment(?:ProcPtr|UPP)|Err|GetPic(?:ProcPtr|UPP)|JShieldCursor(?:ProcPtr|UPP)|Line(?:ProcPtr|UPP)|O(?:pcode(?:ProcPtr|UPP)|val(?:ProcPtr|UPP))|P(?:oly(?:ProcPtr|UPP)|rinterStatus(?:ProcPtr|UPP)|utPic(?:ProcPtr|UPP))|R(?:Rect(?:ProcPtr|UPP)|e(?:ct(?:ProcPtr|UPP)|gionParseDirection)|gn(?:ProcPtr|UPP))|StdGlyphs(?:ProcPtr|UPP)|T(?:ext(?:ProcPtr|UPP)|xMeas(?:ProcPtr|UPP)))|Elem(?:Ptr)?|Hdr(?:Ptr)?|L(?:Preview(?:PDFStyle|RequestRef)|ThumbnailRe(?:f|questRef))|Types)|R(?:DFlagsType|GBColor|OTA(?:GlyphEntry|Header)|TAType|e(?:ad(?:BytesFDF|Packet(?:DataFDF|sFDF))|drawBackground(?:ProcPtr|UPP)|gi(?:onToRects(?:ProcPtr|UPP)|ster(?:Information(?:Intel64|PowerPC)?|edComponent(?:InstanceRecord(?:Ptr)?|Record(?:Ptr)?)))|s(?:Attributes|Err(?:ProcPtr|UPP)|File(?:Attributes|RefNum)|ID|ource(?:Count|EndianFilterPtr|Index|Spec)))|gnHandle|outin(?:e(?:Descriptor(?:Handle|Ptr)?|FlagsType|Record(?:Handle|Ptr)?)|g(?:Flags|Resource(?:Entry|Handle|Ptr)))|srcChainLocation|uleBasedTrslRecord)|S(?:C(?:Bond(?:InterfaceRef|StatusRef)|DynamicStore(?:C(?:allBack|ontext)|Ref)|Network(?:Connection(?:C(?:allBack|ontext)|Flags|PPPStatus|Ref|Status)|InterfaceRef|ProtocolRef|Reachability(?:C(?:allBack|ontext)|Flags|Ref)|Se(?:rviceRef|tRef))|Preferences(?:C(?:allBack|ontext)|Notification|Ref)|VLANInterfaceRef)|FNTLookup(?:ArrayHeader|BinarySearchHeader|FormatSpecificHeader|Kind|Offset|S(?:egment(?:Header)?|ingle(?:Header)?)|T(?:able(?:Format|Handle|Ptr)?|rimmedArrayHeader)|V(?:alue|ectorHeader))|Int|K(?:Document(?:I(?:D|ndexState)|Ref)|Index(?:DocumentIteratorRef|Ref|Type)|S(?:earch(?:GroupRef|Options|Re(?:f|sults(?:FilterCallBack|Ref))|Type)|ummaryRef))|MPTETime(?:Flags|Type)?|R(?:CallBack(?:P(?:aram|rocPtr)|Struct|UPP)|Language(?:Model|Object)|P(?:ath|hrase)|Re(?:cogni(?:tion(?:Result|System)|zer)|jectionLevel)|Spee(?:ch(?:Object|Source)|dSetting)|Word)|SL(?:Authenticate|C(?:ipher(?:Suite|suiteGroup)|lientCertificateState|on(?:nection(?:Ref|Type)|text(?:Ref)?))|Protocol(?:Side)?|ReadFunc|Session(?:Option|State)|WriteFunc)|T(?:Class(?:Table)?|E(?:lement|ntry(?:Index|One|Two|Zero))|H(?:andle|eader)|Ptr|X(?:Class(?:Table)?|Entry(?:Index|One|Two|Zero)|Header|StateIndex))|c(?:hedule(?:dAudio(?:FileRegion(?:CompletionProc)?|Slice(?:CompletionProc)?)|rInfoRec(?:Ptr)?)|r(?:ap(?:Flavor(?:Flags|Info|Type)|PromiseKeeper(?:ProcPtr|UPP)|Ref|T(?:ranslationList(?:Handle|Ptr)?|ype(?:Spec)?))|ipt(?:CodeRun(?:Ptr)?|Language(?:Record|Support(?:Handle|Ptr)?)|TokenType|ingComponentSelector)|oll(?:BarTrackInfo|WindowOptions)|pST(?:Element|Table)))|e(?:c(?:A(?:CLRef|FPServerSignature|ccess(?:Control(?:CreateFlags|Ref)|OwnerType|Ref)|sn1(?:AlgId|Item|Oid|PubKeyInfo|Template(?:Chooser(?:Ptr)?|_struct)?)|uthenticationType)|C(?:S(?:DigestAlgorithm|Flags)|ertificateRef|ode(?:Ref|S(?:ignatureFlags|tatus))|redentialType)|External(?:Format|ItemType)|G(?:roupTransformRef|uestRef)|I(?:dentity(?:Ref|SearchRef)|tem(?:Attr|Class|ImportExport(?:Flags|KeyParameters)))|Key(?:GeneratePairBlock|ImportExport(?:Flags|Parameters)|OperationType|Ref|Sizes|Usage|chain(?:Attr(?:Type|ibute(?:Info|List|Ptr)?)|Callback(?:Info)?|Event(?:Mask)?|ItemRef|PromptSelector|Ref|S(?:e(?:archRef|ttings)|tatus)))|MessageBlock|P(?:a(?:dding|sswordRef)|olicy(?:Ref|SearchRef)|r(?:eferencesDomain|otocolType)|ublicKeyHash)|R(?:andomRef|equirement(?:Ref|Type))|StaticCodeRef|T(?:askRef|r(?:ansform(?:A(?:ctionBlock|ttribute(?:ActionBlock|Ref))|CreateFP|DataBlock|I(?:mplementationRef|nstanceBlock)|MetaAttributeType|Ref|StringOrAttributeRef)|ust(?:Callback|OptionFlags|Re(?:f|sultType)|Settings(?:Domain|KeyUsage|Result)|WithErrorCallback|edApplicationRef)))|uritySessionId)|lectorFunction(?:ProcPtr|UPP)|ssion(?:AttributeBits|CreationFlags)|t(?:PropertyFDF|UserDataFDF|upWindowProxyDragImageRec))|izeResourceRec(?:Handle|Ptr)?|l(?:eepQ(?:ProcPtr|Rec(?:Ptr)?|UPP)|iderTrackInfo)|ound(?:DataChunk(?:Ptr)?|ProcPtr|UPP)|peech(?:Channel(?:Record)?|Done(?:ProcPtr|UPP)|Error(?:CFProcPtr|Info|ProcPtr|UPP)|Phoneme(?:ProcPtr|UPP)|S(?:tatusInfo|ync(?:ProcPtr|UPP))|TextDone(?:ProcPtr|UPP)|VersionInfo|Word(?:CFProcPtr|ProcPtr|UPP)|XtndData)|t(?:Scrp(?:Handle|Ptr|Rec)|a(?:geList|ndard(?:DropLocation|IconListCellData(?:Ptr|Rec)))|ring(?:2DateStatus|ToDateStatus)|yle(?:Run|Table))|ys(?:PPtr|tem(?:SoundID|UI(?:Mode|Options))))|T(?:E(?:C(?:BufferContextRec|Conver(?:sionInfo|terContextRec)|Encoding(?:Pair(?:Rec|s(?:Handle|Ptr|Rec)?)|sList(?:Handle|Ptr|Rec))|In(?:fo(?:Handle|Ptr)?|ternetName(?:Rec|UsageMask|s(?:Handle|Ptr|Rec)))|Locale(?:ListToEncodingList(?:Ptr|Rec)|ToEncodingsList(?:Handle|Ptr|Rec))|ObjectRef|Plugin(?:C(?:lear(?:ContextInfoPtr|SnifferContextInfoPtr)|onvertTextEncodingPtr)|Disp(?:atchTable|oseEncoding(?:ConverterPtr|SnifferPtr))|FlushConversionPtr|Get(?:Count(?:Available(?:SniffersPtr|TextEncoding(?:PairsPtr|sPtr))|DestinationTextEncodingsPtr|MailEncodingsPtr|SubTextEncodingsPtr|WebEncodingsPtr)|PluginDispatchTablePtr|TextEncoding(?:FromInternetNamePtr|InternetNamePtr))|NewEncoding(?:ConverterPtr|SnifferPtr)|S(?:ig(?:nature)?|niffTextEncodingPtr|tateRec)|Version)|S(?:niffer(?:ContextRec|ObjectRef)|ubTextEncoding(?:Rec|s(?:Handle|Ptr|Rec)))|lickLoop(?:ProcPtr|UPP))|DoText(?:ProcPtr|UPP)|FindWord(?:ProcPtr|UPP)|Handle|IntHook|Ptr|Rec(?:alc(?:ProcPtr|UPP))?|Style(?:Handle|Ptr|Rec|Table))|ISInputSourceRef|MTask(?:Ptr)?|S(?:Code|M(?:Doc(?:AccessAttributes|ument(?:I(?:D|nterfaceType)|PropertyTag))|GlyphInfo(?:Array)?)|criptingSizeResource)|X(?:N(?:A(?:TSUI(?:Features|Variations)|ction(?:Key(?:Mapper(?:ProcPtr|UPP))?|NameMapper(?:ProcPtr|UPP))|ttributeData|utoScrollBehavior)|Background(?:Data|Type)?|C(?:arbonEventInfo|o(?:mmandEventSupportOptions|nt(?:extualMenuSetup(?:ProcPtr|UPP)|inuousFlags|rol(?:Data|Tag))|untOptions))|D(?:ataType|rawItems)|Errors|F(?:eatureBits|i(?:leType|nd(?:ProcPtr|UPP))|rame(?:ID|Options|Type))|HyperLinkState|LongRect|Ma(?:rgins|tch(?:Options|TextRecord))|O(?:bject(?:Refcon)?|ffset)|PermanentTextEncodingType|RectKey|Scroll(?:Bar(?:Orientation|State)|Info(?:ProcPtr|UPP)|Unit)|T(?:ab(?:Type)?|ype(?:Attributes|RunAttribute(?:Sizes|s)))|VersionValue)|TNTag)|a(?:ble(?:DirectoryRecord|tP(?:oint(?:Rec|erRec)|roximityRec))|sk(?:Proc|Storage(?:Index|Value)))|ext(?:BreakLocatorRef|Chunk(?:Ptr)?|Encoding(?:Base|Format|NameSelector|R(?:ec|un(?:Ptr)?)|Variant)?|Ptr|Range(?:Array(?:Handle|Ptr)?|Handle|Ptr)?|S(?:ervice(?:Class|Info(?:Ptr)?|List(?:Handle|Ptr)?|Property(?:Tag|Value))|tyle(?:Handle|Ptr)?)|ToUnicodeInfo|WidthHook(?:ProcPtr|UPP))|h(?:eme(?:ArrowOrientation|B(?:ackgroundKind|rush|utton(?:Adornment|Draw(?:Info(?:Ptr)?|ProcPtr|UPP)|Kind|Value))|C(?:heckBoxStyle|ursor)|Dra(?:gSoundKind|w(?:State|ingState))|Erase(?:ProcPtr|UPP)|FontID|GrowDirection|Iterator(?:ProcPtr|UPP)|Me(?:nu(?:BarState|ItemType|State|Type)|tric)|PopupArrowSize|S(?:crollBar(?:ArrowStyle|ThumbStyle)|oundKind)|T(?:ab(?:Direction|Style|TitleDraw(?:ProcPtr|UPP))|extColor|humbDirection|itleBarWidget|rack(?:Attributes|DrawInfo|EnableState|Kind|PressState))|Window(?:Attributes|Metrics(?:Ptr)?|Type))|read(?:Entry(?:ProcPtr|TPP|UPP)|ID|Options|S(?:cheduler(?:ProcPtr|TPP|UPP)|t(?:ate|yle)|witch(?:ProcPtr|TPP|UPP))|T(?:askRef|ermination(?:ProcPtr|TPP|UPP))))|imer(?:ProcPtr|UPP)|o(?:ggle(?:PB|Results)|ken(?:Block(?:Ptr)?|Re(?:c(?:Ptr)?|sults))|olboxObjectClassRef)|r(?:a(?:k(?:Table(?:Data|Entry)?|Value)|ns(?:itionWindowOptions|lation(?:Attributes|Flags|Ref(?:Num)?)))|ipleInt|uncCode)|ype(?:SelectRecord|sBlock(?:Ptr)?))|U(?:AZoomChangeFocusType|C(?:C(?:harProperty(?:Type|Value)|ollat(?:eOptions|ionValue))|Key(?:CharSeq|LayoutFeatureInfo|ModifiersToTableNum|Output|S(?:equenceDataIndex|tate(?:Entry(?:Range|Terminal)|Record(?:sIndex)?|Terminators))|ToCharTableIndex|board(?:Layout|TypeHeader))|T(?:SWalkDirection|extBreak(?:Options|Type)|ypeSelect(?:CompareResult|Options|Ref)))|Int|NDServerRef|RL(?:CallbackInfo|Event(?:Mask)?|Notify(?:ProcPtr|UPP)|OpenFlags|Reference|S(?:tate|ystemEvent(?:ProcPtr|UPP)))|TCDateTime(?:Handle|Ptr)?|n(?:i(?:CharArray(?:Handle|Offset|Ptr)|code(?:Map(?:Version|ping(?:Ptr)?)|ToText(?:Fallback(?:ProcPtr|UPP)|Info|RunInfo)))|tokenTable(?:Handle|Ptr)?)|ser(?:EventUPP|Item(?:ProcPtr|UPP)))|V(?:DGam(?:RecPtr|maRecord)|ector(?:128(?:Intel)?|Information(?:Intel64|PowerPC)?)|o(?:ice(?:Description|FileInfo|Spec(?:Ptr)?)|l(?:MountInfo(?:Header|Ptr)|ume(?:MountInfoHeader(?:Ptr)?|Type))))|W(?:CTab(?:Handle|Ptr)|S(?:ClientContext(?:CopyDescriptionCallBackProcPtr|Re(?:leaseCallBackProcPtr|tainCallBackProcPtr))?|MethodInvocationRef|ProtocolHandler(?:DeserializationProcPtr|Ref|SerializationProcPtr)|TypeID|tateData(?:Handle|Ptr)?)|i(?:d(?:eChar(?:Arr)?|thHook(?:ProcPtr|UPP))|n(?:CTab|dow(?:A(?:ctivationScope|ttributes)|C(?:lass|onstrainOptions)|D(?:ef(?:PartCode|Spec(?:Ptr)?|Type|UPP)|rawerState)|Group(?:Attributes|ContentOptions|Ref)|LatentVisibility|Modality|P(?:a(?:int(?:Proc(?:Options|Ptr)|UPP)|rtCode)|ositionMethod|tr)|Re(?:f|gionCode)|T(?:itleDrawing(?:ProcPtr|UPP)|ransition(?:Action|Effect)))))|ordBreak(?:ProcPtr|UPP)|rit(?:e(?:BytesFDF|PacketsFDF)|ingCode))|XLib(?:ContainerHeader|ExportedSymbol(?:HashSlot|Key)?)|ZoomAcceleration|a(?:cl_(?:entry_(?:id_t|t)|flag(?:_t|set_t)|perm(?:_t|set_(?:mask_t|t))|t(?:ag_t|ype_t)?)|ddr64_t|larm_(?:port_t|t(?:ype_t)?)|rcade_register_t|u(?:_(?:as(?:flgs_t|id_t)|c(?:lass_t|tlmode_t)|e(?:mod_t|v(?:class_map(?:_t)?|ent_t)|xpire_after(?:_t)?)|fstat_t|id_t|mask(?:_t)?|qctrl(?:_t)?|s(?:ession(?:_t)?|tat_t)|t(?:id(?:_(?:addr(?:_t)?|t))?|oken))|dit(?:_(?:fstat|stat|token_t)|info(?:_(?:addr(?:_t)?|t))?|pinfo(?:_(?:addr(?:_t)?|t))?)))|b(?:oo(?:lean_t|tstrap_t)|uild_(?:tool_version|version_command))|c(?:cntTokenRec(?:Handle|Ptr|ord)|lock_(?:attr_t|ctrl_(?:port_t|t)|flavor_t|id_t|re(?:ply_t|s_t)|serv_(?:port_t|t))|msghdr|oalition_t|pu_(?:subtype_t|t(?:hreadtype_t|ype_t))|ssm_(?:a(?:cl_(?:keychain_prompt_selector|process_subject_selector)|pple(?:cspdl_db_(?:change_password_parameters|is_locked_parameters|settings_parameters)|dl_open_parameters(?:_mask)?)|uthorizationgroup)|csp_operational_statistics|d(?:at(?:a|e)|b_schema_index_info|l_(?:db_handle|pkcs11_attributes))|func_name_addr|guid|k(?:ey_size|r_name)|list(?:_element)?|memory_funcs|name_list|parsed_c(?:ert|rl)|query_size_data|range|tp_result_set|version))|d(?:ata_in_code_entry|ec(?:form|imal)|y(?:l(?:d_(?:info_command|kernel_(?:image_info(?:_(?:array_t|t))?|process_info(?:_t)?))|i(?:b(?:_(?:command|module(?:_64)?|reference|table_of_contents))?|nker_command))|symtab_command))|e(?:mulation_vector_t|n(?:cryption_info_command(?:_64)?|try_point_command)|vsio(?:Keymapping|MouseScaling)|x(?:ception_(?:behavior_(?:array_t|t)|data_t(?:ype_t)?|flavor_array_t|handler_(?:array_t|t)|mask_(?:array_t|t)|port_(?:arra(?:ry_t|y_t)|t)|type_t)|tension_data_format))|f(?:e(?:nv_t|xcept_t)|pos_t|vm(?:file_command|lib(?:_command)?))|gpu_energy_data(?:_t)?|h(?:ash_info_bucket(?:_(?:array_t|t))?|ost_(?:basic_info(?:_(?:data_t|t))?|c(?:an_has_debugger_info(?:_(?:data_t|t))?|pu_load_info(?:_(?:data_t|t))?)|flavor_t|info(?:64_t|_(?:data_t|t))|load_info(?:_(?:data_t|t))?|name_(?:port_t|t)|p(?:r(?:eferred_user_arch(?:_(?:data_t|t))?|i(?:ority_info(?:_(?:data_t|t))?|v_t))|urgable_info_(?:data_t|t))|s(?:ched_info(?:_(?:data_t|t))?|ecurity_t)|t))|i(?:386_(?:exception_state_t|float_state_t|thread_state_t)|dent_command|maxdiv_t|nteger_t|o_(?:async_ref(?:64_t|_t)|buf_ptr_t|connect_t|enumerator_t|iterator_t|master_t|name_t|object_t|registry_entry_t|s(?:calar_inband(?:64_t|_t)|ervice_t|t(?:at_(?:entry|info(?:_t)?)|r(?:ing_(?:inband_t|t)|uct_inband_t)))|user_(?:reference_t|scalar_t))|pc_(?:info_(?:name(?:_(?:array_t|t))?|space(?:_(?:basic(?:_t)?|t))?|tree_name(?:_(?:array_t|t))?)|space_(?:inspect_t|port_t|t)|voucher_(?:attr_(?:control_t|manager_t)|t)))|k(?:auth_(?:ac(?:e(?:_(?:rights_t|t))?|l(?:_t)?)|cache_sizes|filesec(?:_t)?|identity_extlookup)|ern(?:_return_t|el_(?:boot_info_t|resource_sizes(?:_(?:data_t|t))?|version_t))|mod_(?:args_t|control_flavor_t|info(?:_(?:32_v1(?:_t)?|64_v1(?:_t)?|array_t|t))?|reference(?:_t)?|st(?:art_func_t|op_func_t)|t)|object_description_t)|l(?:a(?:belstr_t|unch_data_(?:dict_iterator_t|t(?:ype_t)?))|edger_(?:a(?:mount_t|rray_t)|item_t|port_(?:array_t|t)|t)|in(?:ger|ke(?:dit_data_command|r_option_command))|o(?:ad_command|ck(?:_set_(?:port_t|t)|group_info(?:_(?:array_t|t))?)))|m(?:a(?:ch_(?:core_(?:details|fileheader)|dead_name_notification_t|e(?:rror_(?:fn_t|t)|xception_(?:code_t|data_t(?:ype_t)?|subcode_t))|header(?:_64)?|m(?:emory_info(?:_(?:array_t|t))?|sg_(?:audit_trailer_t|b(?:ase_t|its_t|ody_t)|co(?:ntext_trailer_t|py_options_t)|descriptor_t(?:ype_t)?|empty_(?:rcv_t|send_t|t)|format_0_trailer_t|guard(?:_flags_t|ed_port_descriptor(?:32_t|64_t|_t))|header_t|id_t|ma(?:c_trailer_t|x_trailer_t)|o(?:ol_(?:descriptor(?:32_t|64_t|_t)|ports_descriptor(?:32_t|64_t|_t))|ption(?:_t|s_t))|p(?:ort_descriptor_t|riority_t)|return_t|s(?:e(?:curity_trailer_t|qno_trailer_t)|ize_t)|t(?:imeout_t|railer_(?:info_t|size_t|t(?:ype_t)?)|ype_(?:descriptor_t|n(?:ame_t|umber_t)|size_t))))|no_senders_notification_t|port_(?:array_t|context_t|de(?:l(?:eted_notification_t|ta_t)|stroyed_notification_t)|flavor_t|guard_exception_codes|info_(?:ext(?:_t)?|t)|limits(?:_t)?|ms(?:count_t|gcount_t)|name_(?:array_t|t)|options(?:_(?:ptr_t|t))?|qos(?:_t)?|right(?:_t|s_t)|s(?:eqno_t|rights_t|tatus(?:_t)?)|type_(?:array_t|t)|urefs_t)|send_(?:once_notification_t|possible_notification_t)|t(?:ask_basic_info(?:_(?:data_t|t))?|imespec(?:_t)?)|v(?:m_(?:address_t|info_region(?:_t)?|offset_t|read_entry(?:_t)?|size_t)|oucher_(?:attr_(?:co(?:mmand_t|nt(?:ent_(?:size_t|t)|rol_(?:flags_t|t)))|importance_refs|key_(?:array_t|t)|manager_t|r(?:aw_recipe_(?:array_(?:size_t|t)|size_t|t)|ecipe_(?:command_(?:array_t|t)|data(?:_t)?|size_t|t))|value_(?:flags_t|handle_(?:array_(?:size_t|t)|t)|reference_t))|name_(?:array_t|t)|selector_t|t))|zone_(?:info_(?:array_t|data|t)|name(?:_(?:array_t|t))?))|trix_(?:double(?:2x(?:2|3|4)|3x(?:2|3|4)|4x(?:2|3|4))|float(?:2x(?:2|3|4)|3x(?:2|3|4)|4x(?:2|3|4))))|context_t|em(?:_entry_name_port_t|ory_object_(?:a(?:rray_t|ttr_info(?:_(?:data_t|t))?)|behave_info(?:_(?:data_t|t))?|c(?:luster_size_t|o(?:ntrol_t|py_strategy_t))|default_t|f(?:ault_info_t|lavor_t)|info_(?:data_t|t)|name_t|offset_t|perf_info(?:_(?:data_t|t))?|return_t|size_t|t))|ig_(?:impl_routine_t|r(?:eply_error_t|outine_(?:arg_descriptor_t|descriptor(?:_t)?|t))|s(?:erver_routine_t|tub_routine_t|ubsystem(?:_t)?|ymtab(?:_t)?))|sg(?:_labels_t|hdr))|n(?:atural_t|ot(?:e_command|ify_port_t)|space_path_t|tsid_t)|os_(?:block_t|function_t|log_(?:s|t(?:ype_t)?)|trace_payload_(?:object_t|t)|unfair_lock_s)|p(?:a(?:cked_(?:char(?:16|2|32|4|64|8)|double(?:2|4|8)|float(?:16|2|4|8)|int(?:16|2|4|8)|long(?:2|4|8)|short(?:16|2|32|4|8)|u(?:char(?:16|2|32|4|64|8)|int(?:16|2|4|8)|long(?:2|4|8)|short(?:16|2|32|4|8)))|ge_address_array_t)|icker|o(?:inter_t|licy_(?:base(?:_(?:data_t|t)|s)|fifo_(?:base(?:_(?:data_t|t))?|info(?:_(?:data_t|t))?|limit(?:_(?:data_t|t))?)|info(?:_(?:data_t|t)|s)|limit(?:_(?:data_t|t)|s)|rr_(?:base(?:_(?:data_t|t))?|info(?:_(?:data_t|t))?|limit(?:_(?:data_t|t))?)|t(?:imeshare_(?:base(?:_(?:data_t|t))?|info(?:_(?:data_t|t))?|limit(?:_(?:data_t|t))?))?))|pnum_t|r(?:eb(?:ind_cksum_command|ound_dylib_command)|ocessor_(?:array_t|basic_info(?:_(?:data_t|t))?|cpu_load_info(?:_(?:data_t|t))?|flavor_t|info_(?:array_t|data_t|t)|port_(?:array_t|t)|set_(?:array_t|basic_info(?:_(?:data_t|t))?|control_(?:port_t|t)|flavor_t|info_(?:data_t|t)|load_info(?:_(?:data_t|t))?|name_(?:array_t|port_(?:array_t|t)|t)|port_t|t)|t)))|qos_class_t|r(?:e(?:g(?:64_t|isterSelectorType)|lop)|outine(?:_(?:arg_(?:descriptor(?:_t)?|offset|size|type)|descriptor(?:_t)?)|s_command(?:_64)?)|p(?:ath_command|c_(?:routine_(?:arg_descriptor(?:_t)?|descriptor(?:_t)?)|s(?:ignature|ubsystem(?:_t)?))))|s(?:a(?:_endpoints(?:_t)?|e_(?:associd_t|connid_t))|e(?:c(?:_(?:certificate(?:_t)?|identity(?:_t)?|object(?:_t)?|protocol_(?:challenge_(?:complete_t|t)|key_update_(?:complete_t|t)|metadata(?:_t)?|options(?:_t)?|pre_shared_key_selection_(?:complete_t|t)|verify_(?:complete_t|t))|trust(?:_t)?)|tion(?:_64)?|urity_token_t)|gment_command(?:_64)?|maphore_(?:port_t|t))|f(?:_hdtr|nt(?:CMap(?:E(?:ncoding|xtendedSubHeader)|Header|SubHeader)|D(?:escriptorHeader|irectory(?:Entry)?)|F(?:eature(?:Header|Name)|ont(?:Descriptor|FeatureSetting|RunFeature))|Instance|Name(?:Header|Record)|Variation(?:Axis|Header)))|i(?:md_(?:bool|char(?:1(?:6)?|2|3(?:2)?|4|64|8)|double(?:1|2|3|4|8)|float(?:1(?:6)?|2|3|4|8)|int(?:1(?:6)?|2|3|4|8)|long(?:1|2|3|4|8)|packed_(?:char(?:16|2|32|4|64|8)|double(?:2|4|8)|float(?:16|2|4|8)|int(?:16|2|4|8)|long(?:2|4|8)|short(?:16|2|32|4|8)|u(?:char(?:16|2|32|4|64|8)|int(?:16|2|4|8)|long(?:2|4|8)|short(?:16|2|32|4|8)))|short(?:1(?:6)?|2|3(?:2)?|4|8)|u(?:char(?:1(?:6)?|2|3(?:2)?|4|64|8)|int(?:1(?:6)?|2|3|4|8)|long(?:1|2|3|4|8)|short(?:1(?:6)?|2|3(?:2)?|4|8)))|nt(?:16|32|64|8))|leep_type_t|o(?:_np_extensions|ck(?:addr(?:_storage)?|proto)|urce_version_command)|u(?:b_(?:client_command|framework_command|library_command|umbrella_command)|id_cred_(?:path_t|t|uid_t))|y(?:m(?:seg_command|tab_(?:command|name_t))|nc_policy_t))|t(?:ask_(?:a(?:bsolutetime_info(?:_(?:data_t|t))?|ffinity_tag_info(?:_(?:data_t|t))?|rray_t)|basic_info(?:_(?:32(?:_(?:data_t|t))?|64(?:_(?:data_t|t))?|data_t|t))?|category_policy(?:_(?:data_t|t))?|dyld_info(?:_(?:data_t|t))?|e(?:vents_info(?:_(?:data_t|t))?|x(?:c_guard_behavior_t|tmod_info(?:_(?:data_t|t))?))|fla(?:gs_info(?:_(?:data_t|t))?|vor_t)|in(?:fo_(?:data_t|t)|spect_(?:basic_counts(?:_(?:data_t|t))?|flavor(?:_t)?|info_t|t))|kernelmemory_info(?:_(?:data_t|t))?|latency_qos(?:_t)?|name_t|p(?:o(?:licy_(?:flavor_t|t)|rt_(?:array_t|t)|wer_info(?:_(?:data_t|t|v2(?:_(?:data_t|t))?))?)|urgable_info_t)|qos_policy(?:_t)?|role(?:_t)?|s(?:pecial_port_t|uspension_token_t)|t(?:hr(?:ead_times_info(?:_(?:data_t|t))?|oughput_qos(?:_t)?)|race_memory_info(?:_(?:data_t|t))?)?|vm_info(?:_(?:data_t|t))?|wait_state_info(?:_(?:data_t|t))?|zone_info_(?:array_t|data|t))|hread_(?:a(?:ct_(?:array_t|port_(?:array_t|t)|t)|ffinity_policy(?:_(?:data_t|t))?|rray_t)|ba(?:ckground_policy(?:_(?:data_t|t))?|sic_info(?:_(?:data_t|t))?)|command|extended_(?:info(?:_(?:data_t|t))?|policy(?:_(?:data_t|t))?)|flavor_t|i(?:dentifier_info(?:_(?:data_t|t))?|n(?:fo_(?:data_t|t)|spect_t))|latency_qos_(?:policy(?:_(?:data_t|t))?|t)|p(?:o(?:licy_(?:flavor_t|t)|rt_(?:array_t|t))|recedence_policy(?:_(?:data_t|t))?)|sta(?:ndard_policy(?:_(?:data_t|t))?|te_(?:data_t|flavor_(?:array_t|t)|t))|t(?:hroughput_qos_(?:policy(?:_(?:data_t|t))?|t)|ime_constraint_policy(?:_(?:data_t|t))?)?)|ime_value(?:_t)?|l(?:s_(?:ciphersuite_(?:group_t|t)|protocol_version_t)|v_descriptor)|oken_t|wolevel_hint(?:s_command)?)|u(?:ext_object_t|int(?:16|32|64|8)|pl_t|ser_subsystem_t|uid_(?:command|string_t))|v(?:e(?:ctor_(?:char(?:16|2|3(?:2)?|4|8)|double(?:2|3|4|8)|float(?:16|2|3|4|8)|int(?:16|2|3|4|8)|long(?:1|2|3|4|8)|short(?:16|2|3(?:2)?|4|8)|u(?:char(?:16|2|3(?:2)?|4|8)|int(?:16|2|3|4|8)|long(?:1|2|3|4|8)|short(?:16|2|3(?:2)?|4|8)))|rsion_min_command)|fs_path_t|irtual_memory_guard_exception_codes|m(?:32_object_id_t|_(?:address_t|behavior_t|extmod_statistics(?:_(?:data_t|t))?|in(?:fo_(?:object(?:_(?:array_t|t))?|region(?:_(?:64(?:_t)?|t))?)|herit_t)|ma(?:chine_attribute_(?:t|val_t)|p_(?:address_t|offset_t|size_t|t))|named_entry_t|o(?:bject_(?:id_t|offset_t|size_t)|ffset_t)|p(?:age_info_(?:basic(?:_(?:data_t|t))?|data_t|flavor_t|t)|rot_t|urg(?:able_t|eable_(?:info(?:_t)?|stat(?:_t)?)))|re(?:ad_entry(?:_t)?|gion_(?:basic_info(?:_(?:64(?:_t)?|data_(?:64_t|t)|t))?|extended_info(?:_(?:data_t|t))?|flavor_t|info_(?:64_t|data_t|t)|recurse_info_(?:64_t|t)|submap_(?:info(?:_(?:64(?:_t)?|data_(?:64_t|t)|t))?|short_info_(?:64(?:_t)?|data_64_t))|top_info(?:_(?:data_t|t))?))|s(?:ize_t|tatistics(?:64(?:_(?:data_t|t))?|_(?:data_t|t))?|ync_t)|task_entry_t))|o(?:idPtr|ucher_mach_msg_state_(?:s|t)))|x(?:86_(?:avx(?:512_state(?:32_t|64_t|_t)?|_state(?:32_t|64_t|_t)?)|debug_state(?:32_t|64_t|_t)?|exception_state(?:32_t|64_t|_t)?|float_state(?:32_t|64_t|_t)?|pagein_state_t|state_hdr(?:_t)?|thread_(?:full_state64_t|state(?:32_t|64_t|_t)?))|pc_(?:activity_(?:handler_t|state_t|t)|connection_(?:handler_t|t)|endpoint_t|finalizer_t|handler_t|object_t|type_t))|zone_(?:btrecord(?:_(?:array_t|t))?|info(?:_(?:array_t|t))?|name(?:_(?:array_t|t))?))\\b",
+ "name": "support.type.c"
+ },
{
"match": "\\b(?:CF(?:A(?:bsoluteTime|llocator(?:AllocateCallBack|Co(?:ntext|pyDescriptionCallBack)|DeallocateCallBack|PreferredSizeCallBack|Re(?:allocateCallBack|f|leaseCallBack|tainCallBack))|rray(?:ApplierFunction|C(?:allBacks|opyDescriptionCallBack)|EqualCallBack|Re(?:f|leaseCallBack|tainCallBack))|ttributedStringRef)|B(?:ag(?:ApplierFunction|C(?:allBacks|opyDescriptionCallBack)|EqualCallBack|HashCallBack|Re(?:f|leaseCallBack|tainCallBack))|i(?:naryHeap(?:ApplierFunction|C(?:allBacks|ompareContext)|Ref)|t(?:VectorRef)?)|ooleanRef|undleRef(?:Num)?|yteOrder)|C(?:alendar(?:Identifier|Ref|Unit)|haracterSet(?:PredefinedSet|Ref)|ompar(?:atorFunction|isonResult))|D(?:at(?:a(?:Ref|SearchFlags)|e(?:Formatter(?:Key|Ref|Style)|Ref))|ictionary(?:ApplierFunction|CopyDescriptionCallBack|EqualCallBack|HashCallBack|KeyCallBacks|Re(?:f|leaseCallBack|tainCallBack)|ValueCallBacks))|Error(?:Domain|Ref)|File(?:Descriptor(?:C(?:allBack|ontext)|NativeDescriptor|Ref)|Security(?:ClearOptions|Ref))|GregorianUnitFlags|HashCode|I(?:SO8601DateFormatOptions|ndex)|Locale(?:Identifier|Key|LanguageDirection|Ref)|M(?:achPort(?:C(?:allBack|ontext)|InvalidationCallBack|Ref)|essagePort(?:C(?:allBack|ontext)|InvalidationCallBack|Ref)|utable(?:A(?:rrayRef|ttributedStringRef)|B(?:agRef|itVectorRef)|CharacterSetRef|D(?:ataRef|ictionaryRef)|S(?:etRef|tringRef)))|N(?:otification(?:C(?:allback|enterRef)|Name|SuspensionBehavior)|u(?:llRef|mber(?:Formatter(?:Key|OptionFlags|PadPosition|R(?:ef|oundingMode)|Style)|Ref|Type)))|OptionFlags|P(?:lugIn(?:DynamicRegisterFunction|FactoryFunction|Instance(?:DeallocateInstanceDataFunction|GetInterfaceFunction|Ref)|Ref|UnloadFunction)|ropertyList(?:Format|MutabilityOptions|Ref))|R(?:ange|eadStream(?:ClientCallBack|Ref)|unLoop(?:Activity|Mode|Observer(?:C(?:allBack|ontext)|Ref)|R(?:ef|unResult)|Source(?:Context(?:1)?|Ref)|Timer(?:C(?:allBack|ontext)|Ref)))|S(?:et(?:ApplierFunction|C(?:allBacks|opyDescriptionCallBack)|EqualCallBack|HashCallBack|Re(?:f|leaseCallBack|tainCallBack))|ocket(?:C(?:allBack(?:Type)?|ontext)|Error|NativeHandle|Ref|Signature)|tr(?:eam(?:ClientContext|E(?:rror(?:Domain)?|ventType)|PropertyKey|Status)|ing(?:BuiltInEncodings|CompareFlags|Encoding(?:s)?|InlineBuffer|NormalizationForm|Ref|Tokenizer(?:Ref|TokenType)))|wappedFloat(?:32|64))|T(?:ime(?:Interval|Zone(?:NameStyle|Ref))|ree(?:ApplierFunction|Co(?:ntext|pyDescriptionCallBack)|Re(?:f|leaseCallBack|tainCallBack))|ype(?:ID|Ref))|U(?:RL(?:Bookmark(?:CreationOptions|FileCreationOptions|ResolutionOptions)|ComponentType|E(?:numerator(?:Options|Re(?:f|sult))|rror)|PathStyle|Ref)|UID(?:Bytes|Ref)|serNotification(?:CallBack|Ref))|WriteStream(?:ClientCallBack|Ref)|XML(?:Attribute(?:DeclarationInfo|ListDeclarationInfo)|Document(?:Info|TypeInfo)|E(?:lement(?:Info|TypeDeclarationInfo)|ntity(?:Info|ReferenceInfo|TypeCode)|xternalID)|No(?:de(?:Ref|TypeCode)|tationInfo)|P(?:arser(?:AddChildCallBack|C(?:allBacks|o(?:ntext|pyDescriptionCallBack)|reateXMLStructureCallBack)|EndXMLStructureCallBack|HandleErrorCallBack|Options|Re(?:f|leaseCallBack|solveExternalEntityCallBack|tainCallBack)|StatusCode)|rocessingInstructionInfo)|TreeRef))|FSRef)\\b",
"name": "support.type.cf.c"
},
{
- "match": "\\b(?:FILE|accessx_descriptor|blk(?:cnt_t|size_t)|c(?:addr_t|lock(?:_t|id_t)|t_rune_t)|d(?:addr_t|ev_t|i(?:spatch_time_t|v_t)|ouble_t)|errno_t|f(?:bootstraptransfer(?:_t)?|c(?:hecklv(?:_t)?|odeblobs(?:_t)?)|d_(?:mask|set)|i(?:lesec_(?:property_t|t)|xpt_t)|lo(?:at_t|ck(?:timeout)?)|pos_t|s(?:blkcnt_t|filcnt_t|i(?:d(?:_t)?|gnatures(?:_t)?)|obj_id(?:_t)?|searchblock|tore(?:_t)?))|g(?:id_t|uid_t)|i(?:d(?:_t|type_t)|n(?:_(?:addr_t|port_t)|o(?:64_t|_t)|t(?:16_t|32_t|64_t|8_t|_(?:fast(?:16_t|32_t|64_t|8_t)|least(?:16_t|32_t|64_t|8_t))|max_t|ptr_t)))|jmp_buf|key_t|l(?:conv|div_t|ldiv_t|og2phys)|m(?:ach_port_t|ode_t)|nlink_t|off_t|p(?:id_t|roc_rlimit_control_wakeupmon|trdiff_t)|q(?:addr_t|uad_t)|r(?:advisory|egister_t|lim(?:_t|it)|size_t|u(?:ne_t|sage(?:_info_(?:current|t|v(?:0|1|2|3)))?))|s(?:e(?:archstate|gsz_t)|i(?:g(?:_(?:atomic_t|t)|action|event|info_t|jmp_buf|s(?:et_t|tack)|vec)|ze_t)|size_t|tack_t|useconds_t|wblk_t|yscall_arg_t)|t(?:ime(?:_t|spec|val)|m)|u(?:_(?:char|int(?:16_t|32_t|64_t|8_t)?|long|quad_t|short)|context_t|i(?:d_t|nt(?:16_t|32_t|64_t|8_t|_(?:fast(?:16_t|32_t|64_t|8_t)|least(?:16_t|32_t|64_t|8_t))|max_t|ptr_t)?)|s(?:e(?:conds_t|r_(?:addr_t|long_t|off_t|s(?:ize_t|size_t)|time_t|ulong_t))|hort)|uid_t)|va_list|wint_t)\\b",
+ "match": "\\b(?:accessx_descriptor|blk(?:cnt_t|size_t)|c(?:addr_t|lock(?:_t|i(?:d_t|nfo))|t_rune_t)|d(?:addr_t|ev_t|i(?:spatch_time_t|v_t)|ouble_t)|e(?:rrno_t|xception)|f(?:bootstraptransfer(?:_t)?|c(?:hecklv(?:_t)?|odeblobs(?:_t)?)|d_(?:mask|set)|i(?:lesec_(?:property_t|t)|xpt_t)|lo(?:at_t|ck(?:timeout)?)|punchhole(?:_t)?|s(?:blkcnt_t|filcnt_t|i(?:d(?:_t)?|gnatures(?:_t)?)|obj_id(?:_t)?|pecread(?:_t)?|searchblock|tore(?:_t)?)|trimactivefile(?:_t)?)|g(?:id_t|uid_t)|i(?:d(?:_t|type_t)|n(?:_(?:addr_t|port_t)|o(?:64_t|_t)|t(?:16_t|32_t|64_t|8_t|_(?:fast(?:16_t|32_t|64_t|8_t)|least(?:16_t|32_t|64_t|8_t))|max_t|ptr_t))|ovec|timerval)|jmp_buf|key_t|l(?:conv|div_t|ldiv_t|og2phys)|m(?:a(?:ch_port_t|x_align_t)|ode_t)|nlink_t|off_t|p(?:id_t|roc_rlimit_control_wakeupmon|trdiff_t)|q(?:addr_t|uad_t)|r(?:advisory|egister_t|lim(?:_t|it)|size_t|u(?:ne_t|sage(?:_info_(?:current|t|v(?:0|1|2|3|4)))?))|s(?:a_family_t|e(?:archstate|gsz_t)|i(?:g(?:_(?:atomic_t|t)|action|event|info_t|jmp_buf|s(?:et_t|tack)|vec)|md_(?:double(?:2x(?:2|3|4)|3x(?:2|3|4)|4x(?:2|3|4))|float(?:2x(?:2|3|4)|3x(?:2|3|4)|4x(?:2|3|4))|quat(?:d|f))|ze_t)|ocklen_t|size_t|tack_t|useconds_t|wblk_t|yscall_arg_t)|t(?:ime(?:_t|spec|val(?:64)?|zone)|m)|u(?:_(?:char|int(?:16_t|32_t|64_t|8_t)?|long|quad_t|short)|context_t|i(?:d_t|nt(?:16_t|32_t|64_t|8_t|_(?:fast(?:16_t|32_t|64_t|8_t)|least(?:16_t|32_t|64_t|8_t))|max_t|ptr_t)?)|s(?:e(?:conds_t|r_(?:addr_t|long_t|off_t|s(?:ize_t|size_t)|time_t|ulong_t))|hort)|uid_t)|va_list|w(?:char_t|int_t))\\b",
"name": "support.type.clib.c"
},
{
- "match": "\\bdispatch_(?:autorelease_frequency_t|block_(?:flags_t|t)|data_(?:applier_t|s|t)|f(?:d_t|unction_t)|group_(?:s|t)|io_(?:close_flags_t|handler_t|interval_flags_t|s|t(?:ype_t)?)|o(?:bject_(?:s|t)|nce_t)|q(?:os_class_t|ueue_(?:attr_(?:s|t)|priority_t|s|t))|s(?:emaphore_(?:s|t)|ource_(?:m(?:ach_send_flags_t|emorypressure_flags_t)|proc_flags_t|s|t(?:imer_flags_t|ype_(?:s|t))?|vnode_flags_t)))\\b",
+ "match": "\\bdispatch_(?:autorelease_frequency_t|block_(?:flags_t|t)|channel_s|data_(?:s|t)|f(?:d_t|unction_t)|group_(?:s|t)|io_(?:close_flags_t|handler_t|interval_flags_t|s|t(?:ype_t)?)|mach_(?:msg_s|s)|o(?:bject_(?:s|t)|nce_t)|q(?:os_class_t|ueue_(?:attr_(?:s|t)|concurrent_t|global_t|main_t|priority_t|s(?:erial_t)?|t))|s(?:emaphore_(?:s|t)|ource_(?:m(?:ach_(?:recv_flags_t|send_flags_t)|emorypressure_flags_t)|proc_flags_t|s|t(?:imer_flags_t|ype_(?:s|t))?|vnode_flags_t))|workloop_t)\\b",
"name": "support.type.dispatch.c"
},
{
@@ -110,16 +290,52 @@
"name": "support.type.mac-classic.c"
},
{
- "match": "\\b(?:pthread_(?:attr_t|cond(?:_t|attr_t)|key_t|mutex(?:_t|attr_t)|o(?:nce_t|verride_(?:s|t))|rwlock(?:_t|attr_t)|t)|sched_param)\\b",
+ "match": "\\bpthread_(?:attr_t|cond(?:_t|attr_t)|key_t|mutex(?:_t|attr_t)|once_t|rwlock(?:_t|attr_t)|t)\\b",
"name": "support.type.pthread.c"
},
{
- "match": "\\bCGImageByteOrderInfo\\b",
- "name": "support.type.quartz.10.12.c"
+ "match": "\\b(?:CG(?:AffineTransform|B(?:itmap(?:ContextReleaseDataCallback|Info)|lendMode|uttonCount)|C(?:aptureOptions|harCode|o(?:lor(?:ConversionInfo(?:Ref|TransformType)?|Re(?:f|nderingIntent)|Space(?:Model|Ref)?)?|n(?:figureOption|text(?:Ref)?)))|D(?:ata(?:Consumer(?:Callbacks|PutBytesCallback|Re(?:f|leaseInfoCallback))?|Provider(?:DirectCallbacks|GetByte(?:PointerCallback|s(?:AtPositionCallback|Callback))|Re(?:f|lease(?:BytePointerCallback|DataCallback|InfoCallback)|windCallback)|S(?:equentialCallbacks|kipForwardCallback))?)|eviceColor|i(?:rectDisplayID|splay(?:BlendFraction|C(?:hangeSummaryFlags|o(?:nfigRef|unt))|Err|Fade(?:Interval|ReservationToken)|Mode(?:Ref)?|Re(?:configurationCallBack|servationInterval)|Stream(?:Frame(?:AvailableHandler|Status)|Ref|Update(?:Re(?:ctType|f))?)?)))|E(?:rror|vent(?:Err|F(?:i(?:eld|lterMask)|lags)|M(?:ask|ouseSubtype)|Ref|S(?:ource(?:KeyboardType|Ref|StateID)|uppressionState)|T(?:ap(?:CallBack|Information|Location|Options|P(?:lacement|roxy))|imestamp|ype)))|F(?:loat|ont(?:Index|PostScriptFormat|Ref)?|unction(?:Callbacks|EvaluateCallback|Re(?:f|leaseInfoCallback))?)|G(?:ammaValue|esturePhase|lyph(?:DeprecatedEnum)?|radient(?:DrawingOptions|Ref)?)|I(?:mage(?:AlphaInfo|ByteOrderInfo|PixelFormatInfo|Ref)?|nterpolationQuality)|KeyCode|L(?:ayer(?:Ref)?|ine(?:Cap|Join))|M(?:o(?:mentumScrollPhase|useButton)|utablePathRef)|OpenGLDisplayMask|P(?:DF(?:A(?:ccessPermissions|rray(?:Ref)?)|Bo(?:olean|x)|ContentStream(?:Ref)?|D(?:ataFormat|ictionary(?:ApplierFunction|Ref)?|ocument(?:Ref)?)|Integer|O(?:bject(?:Ref|Type)?|perator(?:Callback|Table(?:Ref)?))|Page(?:Ref)?|Real|S(?:canner(?:Ref)?|tr(?:eam(?:Ref)?|ing(?:Ref)?))|Tag(?:Property|Type))|SConverter(?:Begin(?:DocumentCallback|PageCallback)|Callbacks|End(?:DocumentCallback|PageCallback)|MessageCallback|ProgressCallback|Re(?:f|leaseInfoCallback))?|at(?:h(?:Appl(?:ierFunction|yBlock)|DrawingMode|Element(?:Type)?|Ref)?|tern(?:Callbacks|DrawPatternCallback|Re(?:f|leaseInfoCallback)|Tiling)?)|oint)|Re(?:ct(?:Count|Edge)?|freshRate)|S(?:cr(?:een(?:RefreshCallback|Update(?:Move(?:Callback|Delta)|Operation))|oll(?:EventUnit|Phase))|hading(?:Ref)?|ize)|Text(?:DrawingMode|Encoding)|Vector|W(?:heelCount|indow(?:BackingType|I(?:D|mageOption)|L(?:evel(?:Key)?|istOption)|SharingType)))|IOSurfaceRef)\\b",
+ "name": "support.type.quartz.c"
},
{
- "match": "\\b(?:CG(?:AffineTransform|B(?:itmap(?:ContextReleaseDataCallback|Info)|lendMode|uttonCount)|C(?:aptureOptions|harCode|o(?:lor(?:ConversionInfo(?:Ref|TransformType)?|Re(?:f|nderingIntent)|Space(?:Model|Ref)?)?|n(?:figureOption|text(?:Ref)?)))|D(?:ata(?:Consumer(?:Callbacks|PutBytesCallback|Re(?:f|leaseInfoCallback))?|Provider(?:DirectCallbacks|GetByte(?:PointerCallback|s(?:AtPositionCallback|Callback))|Re(?:f|lease(?:BytePointerCallback|DataCallback|InfoCallback)|windCallback)|S(?:equentialCallbacks|kipForwardCallback))?)|eviceColor|i(?:rectDisplayID|splay(?:BlendFraction|C(?:hangeSummaryFlags|o(?:nfigRef|unt))|Err|Fade(?:Interval|ReservationToken)|Mode(?:Ref)?|Re(?:configurationCallBack|servationInterval)|Stream(?:Frame(?:AvailableHandler|Status)|Ref|Update(?:Re(?:ctType|f))?)?)))|E(?:rror|vent(?:Err|F(?:i(?:eld|lterMask)|lags)|M(?:ask|ouseSubtype)|Ref|S(?:ource(?:KeyboardType|Ref|StateID)|uppressionState)|T(?:ap(?:CallBack|Information|Location|Options|P(?:lacement|roxy))|imestamp|ype)))|F(?:loat|ont(?:Index|PostScriptFormat|Ref)?|unction(?:Callbacks|EvaluateCallback|Re(?:f|leaseInfoCallback))?)|G(?:ammaValue|esturePhase|lyph(?:DeprecatedEnum)?|radient(?:DrawingOptions|Ref)?)|I(?:mage(?:AlphaInfo|ByteOrderInfo|Ref)?|nterpolationQuality)|KeyCode|L(?:ayer(?:Ref)?|ine(?:Cap|Join))|M(?:o(?:mentumScrollPhase|useButton)|utablePathRef)|OpenGLDisplayMask|P(?:DF(?:Array(?:Ref)?|Bo(?:olean|x)|ContentStream(?:Ref)?|D(?:ataFormat|ictionary(?:ApplierFunction|Ref)?|ocument(?:Ref)?)|Integer|O(?:bject(?:Ref|Type)?|perator(?:Callback|Table(?:Ref)?))|Page(?:Ref)?|Real|S(?:canner(?:Ref)?|tr(?:eam(?:Ref)?|ing(?:Ref)?)))|SConverter(?:Begin(?:DocumentCallback|PageCallback)|Callbacks|End(?:DocumentCallback|PageCallback)|MessageCallback|ProgressCallback|Re(?:f|leaseInfoCallback))?|at(?:h(?:ApplierFunction|DrawingMode|Element(?:Type)?|Ref)?|tern(?:Callbacks|DrawPatternCallback|Re(?:f|leaseInfoCallback)|Tiling)?)|oint)|Re(?:ct(?:Count|Edge)?|freshRate)|S(?:cr(?:een(?:RefreshCallback|Update(?:Move(?:Callback|Delta)|Operation))|oll(?:EventUnit|Phase))|hading(?:Ref)?|ize)|Text(?:DrawingMode|Encoding)|Vector|W(?:heelCount|indow(?:BackingType|I(?:D|mageOption)|L(?:evel(?:Key)?|istOption)|SharingType)))|IOSurfaceRef)\\b",
- "name": "support.type.quartz.c"
+ "match": "\\b(?:k(?:C(?:FHTTPVersion2_0|GImage(?:Destination(?:EmbedThumbnail|ImageMaxPixelSize)|MetadataShouldExcludeGPS|Property(?:8BIMVersion|APNG(?:DelayTime|LoopCount|UnclampedDelayTime)|GPSHPositioningError|MakerAppleDictionary))|T(?:FontOpenTypeFeature(?:Tag|Value)|RubyAnnotationAttributeName)|V(?:ImageBufferAlphaChannelIsOpaque|PixelFormatCo(?:mponentRange(?:_(?:FullRange|VideoRange|WideRange))?|ntains(?:RGB|YCbCr))))|Sec(?:AttrAccess(?:Control|ibleWhenPasscodeSetThisDeviceOnly)|UseOperationPrompt)|UTType(?:3DContent|A(?:VIMovie|pple(?:ProtectedMPEG4Video|Script)|ssemblyLanguageSource|udioInterchangeFileFormat)|B(?:inaryPropertyList|ookmark|zip2Archive)|C(?:alendarEvent|ommaSeparatedText)|DelimitedText|E(?:lectronicPublication|mailMessage)|Font|GNUZipArchive|InternetLocation|J(?:SON|ava(?:Archive|Class|Script))|Log|M(?:3UPlaylist|IDIAudio|PEG2(?:TransportStream|Video))|OSAScript(?:Bundle)?|P(?:HPScript|KCS12|erlScript|l(?:aylist|uginBundle)|r(?:esentation|opertyList)|ythonScript)|QuickLookGenerator|R(?:awImage|ubyScript)|S(?:c(?:alableVectorGraphics|ript)|hellScript|p(?:otlightImporter|readsheet)|ystemPreferencesPane)|T(?:abSeparatedText|oDoItem)|U(?:RLBookmarkData|TF8TabSeparatedText|nixExecutable)|W(?:aveformAudio|indowsExecutable)|X(?:509Certificate|MLPropertyList|PCService)|ZipArchive))|matrix_identity_(?:double(?:2x2|3x3|4x4)|float(?:2x2|3x3|4x4)))\\b",
+ "name": "support.variable.10.10.c"
+ },
+ {
+ "match": "\\bk(?:A(?:XListItem(?:IndexTextAttribute|LevelTextAttribute|PrefixTextAttribute)|udioComponent(?:InstanceInvalidationNotification|RegistrationsChangedNotification))|C(?:FStreamPropertySocketExtendedBackgroundIdleMode|GImage(?:Property(?:ExifSubsecTimeOriginal|PNGCompressionFilter|TIFFTile(?:Length|Width))|SourceSubsampleFactor)|V(?:ImageBuffer(?:ColorPrimaries_(?:DCI_P3|ITU_R_2020|P3_D65)|TransferFunction_ITU_R_2020|YCbCrMatrix_(?:DCI_P3|ITU_R_2020|P3_D65))|MetalTextureCacheMaximumTextureAgeKey|PixelBuffer(?:MetalCompatibilityKey|OpenGLTextureCacheCompatibilityKey)))|MDItemHTMLContent|Sec(?:A(?:CLAuthorization(?:Integrity|PartitionID)|ttrSyncViewHint)|PolicyApplePayIssuerEncryption|TrustCertificateTransparency|UseAuthentication(?:Context|UI(?:Allow|Fail|Skip)?))|UTTypeSwiftSource)\\b",
+ "name": "support.variable.10.11.c"
+ },
+ {
+ "match": "\\bk(?:C(?:FStreamNetworkServiceTypeCallSignaling|GImage(?:DestinationOptimizeColorForSharing|PropertyDNG(?:AsShot(?:Neutral|WhiteXY)|B(?:aseline(?:Exposure|Noise|Sharpness)|lackLevel)|C(?:a(?:librationIlluminant(?:1|2)|meraCalibration(?:1|2|Signature))|olorMatrix(?:1|2))|FixVignetteRadial|NoiseProfile|Pr(?:ivateData|ofileCalibrationSignature)|W(?:arp(?:Fisheye|Rectilinear)|hiteLevel)))|T(?:BackgroundColorAttributeName|FontDownloadedAttribute|HorizontalInVerticalFormsAttributeName|RubyAnnotationS(?:caleToFitAttributeName|izeFactorAttributeName)|TrackingAttributeName)|VImageBufferTransferFunction_SMPTE_ST_428_1)|IOSurfacePixelSizeCastingAllowed|Sec(?:Attr(?:AccessGroupToken|KeyTypeECSECPrimeRandom|TokenID(?:SecureEnclave)?)|Key(?:Algorithm(?:EC(?:D(?:HKeyExchange(?:Cofactor(?:X963SHA(?:1|2(?:24|56)|384|512))?|Standard(?:X963SHA(?:1|2(?:24|56)|384|512))?)|SASignature(?:DigestX962(?:SHA(?:1|2(?:24|56)|384|512))?|MessageX962SHA(?:1|2(?:24|56)|384|512)|RFC4754))|IESEncryption(?:CofactorX963SHA(?:1AESGCM|2(?:24AESGCM|56AESGCM)|384AESGCM|512AESGCM)|StandardX963SHA(?:1AESGCM|2(?:24AESGCM|56AESGCM)|384AESGCM|512AESGCM)))|RSA(?:Encryption(?:OAEPSHA(?:1(?:AESGCM)?|2(?:24(?:AESGCM)?|56(?:AESGCM)?)|384(?:AESGCM)?|512(?:AESGCM)?)|PKCS1|Raw)|Signature(?:DigestPKCS1v15(?:Raw|SHA(?:1|2(?:24|56)|384|512))|MessagePKCS1v15SHA(?:1|2(?:24|56)|384|512)|Raw)))|KeyExchangeParameter(?:RequestedSize|SharedInfo)))|UTTypeLivePhoto)\\b",
+ "name": "support.variable.10.12.c"
+ },
+ {
+ "match": "\\bk(?:C(?:GImage(?:AuxiliaryData(?:Info(?:Data(?:Description)?|Metadata)|TypeD(?:epth|isparity))|Metadata(?:NamespaceIPTCExtension|PrefixIPTCExtension)|Property(?:AuxiliaryData(?:Type)?|BytesPerRow|FileContentsDictionary|Height|I(?:PTCExt(?:A(?:boutCvTerm(?:CvId|Id|Name|RefinedAbout)?|ddlModelInfo|rtwork(?:C(?:ircaDateCreated|o(?:nt(?:entDescription|ributionDescription)|pyright(?:Notice|Owner(?:ID|Name)))|reator(?:ID)?)|DateCreated|Licensor(?:ID|Name)|OrObject|PhysicalDescription|S(?:ource(?:Inv(?:URL|entoryNo))?|tylePeriod)|Title)|udio(?:Bitrate(?:Mode)?|ChannelCount))|C(?:ircaDateCreated|o(?:nt(?:ainerFormat(?:Identifier|Name)?|r(?:ibutor(?:Identifier|Name|Role)?|olledVocabularyTerm))|pyrightYear)|reator(?:Identifier|Name|Role)?)|D(?:ataOnScreen(?:Region(?:D|H|Text|Unit|W|X|Y)?)?|igital(?:ImageGUID|Source(?:FileType|Type))|opesheet(?:Link(?:Link(?:Qualifier)?)?)?)|E(?:mb(?:dEncRightsExpr|eddedEncodedRightsExpr(?:LangID|Type)?)|pisode(?:Identifier|N(?:ame|umber))?|vent|xternalMetadataLink)|FeedIdentifier|Genre(?:Cv(?:Id|Term(?:Id|Name|RefinedAbout)))?|Headline|IPTCLastEdited|L(?:inkedEnc(?:RightsExpr|odedRightsExpr(?:LangID|Type)?)|ocation(?:C(?:ity|ountry(?:Code|Name)|reated)|GPS(?:Altitude|L(?:atitude|ongitude))|Identifier|Location(?:Id|Name)|ProvinceState|S(?:hown|ublocation)|WorldRegion))|M(?:axAvail(?:Height|Width)|odelAge)|OrganisationInImage(?:Code|Name)|P(?:erson(?:Heard(?:Identifier|Name)?|InImage(?:C(?:haracteristic|vTerm(?:CvId|Id|Name|RefinedAbout))|Description|Id|Name|WDetails)?)|roductInImage(?:Description|GTIN|Name)?|ublicationEvent(?:Date|Identifier|Name)?)|R(?:ating(?:R(?:atingRegion|egion(?:C(?:ity|ountry(?:Code|Name))|GPS(?:Altitude|L(?:atitude|ongitude))|Identifier|Location(?:Id|Name)|ProvinceState|Sublocation|WorldRegion))|S(?:caleM(?:axValue|inValue)|ourceLink)|Value(?:LogoLink)?)?|e(?:gistry(?:EntryRole|I(?:D|temID)|OrganisationID)|leaseReady))|S(?:e(?:ason(?:Identifier|N(?:ame|umber))?|ries(?:Identifier|Name)?)|hownEvent(?:Identifier|Name)?|t(?:orylineIdentifier|reamReady|ylePeriod)|upplyChainSource(?:Identifier|Name)?)|T(?:emporalCoverage(?:From|To)?|ranscript(?:Link(?:Link(?:Qualifier)?)?)?)|Vi(?:deo(?:Bitrate(?:Mode)?|DisplayAspectRatio|EncodingProfile|S(?:hotType(?:Identifier|Name)?|treamsCount))|sualColor)|WorkflowTag(?:Cv(?:Id|Term(?:Id|Name|RefinedAbout)))?)|mage(?:Count|s))|NamedColorSpace|P(?:ixelFormat|rimaryImage)|ThumbnailImages|Width))|T(?:BaselineOffsetAttributeName|FontVariationAxisHiddenKey)|V(?:ImageBuffer(?:ContentLightLevelInfoKey|MasteringDisplayColorVolumeKey|TransferFunction_(?:ITU_R_2100_HLG|SMPTE_ST_2084_PQ|sRGB))|MetalTextureUsage))|IOSurface(?:Plane(?:BitsPerElement|Component(?:Bit(?:Depths|Offsets)|Names|Ranges|Types))|Subsampling)|Sec(?:A(?:CLAuthorizationChange(?:ACL|Owner)|ttrPersist(?:antReference|entReference))|KeyAlgorithm(?:ECIESEncryption(?:CofactorVariableIVX963SHA(?:2(?:24AESGCM|56AESGCM)|384AESGCM|512AESGCM)|StandardVariableIVX963SHA(?:2(?:24AESGCM|56AESGCM)|384AESGCM|512AESGCM))|RSASignature(?:DigestPSSSHA(?:1|2(?:24|56)|384|512)|MessagePSSSHA(?:1|2(?:24|56)|384|512)))))\\b",
+ "name": "support.variable.10.13.c"
+ },
+ {
+ "match": "\\bkC(?:GImage(?:AuxiliaryDataTypePortraitEffectsMatte|Property(?:DNG(?:A(?:ctiveArea|n(?:alogBalance|tiAliasStrength)|sShot(?:ICCProfile|Pr(?:eProfileMatrix|ofileName)))|B(?:a(?:selineExposureOffset|yerGreenSplit)|estQualityScale|lackLevel(?:Delta(?:H|V)|RepeatDim))|C(?:FA(?:Layout|PlaneColor)|hromaBlurRadius|olorimetricReference|urrent(?:ICCProfile|PreProfileMatrix))|Default(?:BlackRender|Crop(?:Origin|Size)|Scale|UserCrop)|ExtraCameraProfiles|ForwardMatrix(?:1|2)|Linear(?:ResponseLimit|izationTable)|Ma(?:kerNoteSafety|skedAreas)|N(?:ewRawImageDigest|oiseReductionApplied)|O(?:pcodeList(?:1|2|3)|riginal(?:BestQualityFinalSize|Default(?:CropSize|FinalSize)|RawFile(?:D(?:ata|igest)|Name)))|Pr(?:eview(?:Application(?:Name|Version)|ColorSpace|DateTime|Settings(?:Digest|Name))|ofile(?:Copyright|EmbedPolicy|HueSatMap(?:D(?:ata(?:1|2)|ims)|Encoding)|LookTable(?:D(?:ata|ims)|Encoding)|Name|ToneCurve))|R(?:aw(?:DataUniqueID|ImageDigest|ToPreviewGain)|eductionMatrix(?:1|2)|owInterleaveFactor)|S(?:hadowScale|ubTileBlockSize))|PNG(?:Comment|Disclaimer|Source|Warning)))|TTypesetterOptionAllowUnboundedLayout|V(?:ImageBufferTransferFunction_Linear|PixelFormatContainsGrayscale))\\b",
+ "name": "support.variable.10.14.c"
+ },
+ {
+ "match": "\\bk(?:C(?:FStreamProperty(?:Allow(?:ConstrainedNetworkAccess|ExpensiveNetworkAccess)|ConnectionIsExpensive)|GImage(?:A(?:nimation(?:DelayTime|LoopCount|StartIndex)|uxiliaryDataTypeSemanticSegmentation(?:HairMatte|SkinMatte|TeethMatte))|Property(?:APNG(?:CanvasPixel(?:Height|Width)|FrameInfoArray)|Exif(?:CompositeImage|OffsetTime(?:Digitized|Original)?|Source(?:ExposureTimesOfCompositeImage|ImageNumberOfCompositeImage))|GIF(?:CanvasPixel(?:Height|Width)|FrameInfoArray)|HEICS(?:CanvasPixel(?:Height|Width)|D(?:elayTime|ictionary)|FrameInfoArray|LoopCount|UnclampedDelayTime)))|TFontFeature(?:SampleTextKey|TooltipTextKey)|V(?:ImageBufferAlphaChannelMode(?:Key|_(?:PremultipliedAlpha|StraightAlpha))|MetalTextureStorageMode))|MIDIPropertyNameConfigurationDictionary|Sec(?:PropertyType(?:Array|Number)|UseDataProtectionKeychain))\\b",
+ "name": "support.variable.10.15.c"
+ },
+ {
+ "match": "\\bkColorSyncExtendedRange\\b",
+ "name": "support.variable.10.16.c"
+ },
+ {
+ "match": "\\bk(?:C(?:FStream(?:NetworkServiceType(?:AVStreaming|Responsive(?:AV|Data))|Property(?:ConnectionIsCellular|NoCellular))|GImage(?:Destination(?:DateTime|Me(?:rgeMetadata|tadata)|Orientation)|Metadata(?:EnumerateRecursively|Namespace(?:DublinCore|Exif(?:Aux)?|IPTCCore|Photoshop|TIFF|XMP(?:Basic|Rights))|Prefix(?:DublinCore|Exif(?:Aux)?|IPTCCore|Photoshop|TIFF|XMP(?:Basic|Rights))|ShouldExcludeXMP))|T(?:Baseline(?:Class(?:AttributeName|Hanging|Ideographic(?:Centered|High|Low)|Math|Roman)|InfoAttributeName|OriginalFont|Reference(?:Font|InfoAttributeName))|FontD(?:escriptorMatching(?:CurrentAssetSize|Descriptors|Error|Percentage|Result|SourceDescriptor|Total(?:AssetSize|DownloadedSize))|ownloadableAttribute)|WritingDirectionAttributeName)|VImageBufferColorPrimaries_P22)|Sec(?:O(?:AEP(?:EncodingParametersAttributeName|M(?:GF1DigestAlgorithmAttributeName|essageLengthAttributeName))|IDSRVName)|P(?:addingOAEPKey|olicyAppleTimeStamping|rivateKeyAttrs|ublicKeyAttrs)))\\b",
+ "name": "support.variable.10.8.c"
+ },
+ {
+ "match": "\\b(?:XPC_ACTIVITY_(?:ALLOW_BATTERY|CHECK_IN|DELAY|GRACE_PERIOD|INTERVAL(?:_(?:1(?:5_MIN|_(?:DAY|HOUR|MIN))|30_MIN|4_HOURS|5_MIN|7_DAYS|8_HOURS))?|PRIORITY(?:_(?:MAINTENANCE|UTILITY))?|RE(?:PEATING|QUIRE_SCREEN_SLEEP))|k(?:AX(?:MarkedMisspelledTextAttribute|TrustedCheckOptionPrompt)|C(?:FStreamPropertySSLContext|GImage(?:Metadata(?:NamespaceExifEX|PrefixExifEX)|Property(?:Exif(?:ISOSpeed(?:Latitude(?:yyy|zzz))?|RecommendedExposureIndex|S(?:ensitivityType|tandardOutputSensitivity))|OpenEXR(?:AspectRatio|Dictionary))|SourceShouldCacheImmediately)|TLanguageAttributeName)|S(?:ec(?:Attr(?:Access(?:Group|ible(?:AfterFirstUnlock(?:ThisDeviceOnly)?|WhenUnlocked(?:ThisDeviceOnly)?)?)|KeyTypeEC|Synchronizable(?:Any)?)|Policy(?:Apple(?:PassbookSigning|Revocation)|RevocationFlags|TeamIdentifier)|Trust(?:E(?:valuationDate|xtendedValidation)|OrganizationName|Re(?:sultValue|vocation(?:Checked|ValidUntilDate))))|peech(?:AudioOutputFormatProperty|Output(?:ChannelMapProperty|ToFileDescriptorProperty)|SynthExtensionProperty)))|vm_kernel_page_(?:mask|s(?:hift|ize)))\\b",
+ "name": "support.variable.10.9.c"
+ },
+ {
+ "match": "\\b(?:CATransform3DIdentity|KERNEL_(?:AUDIT_TOKEN|SECURITY_TOKEN)|NDR_record|UUID_NULL|bootstrap_port|gGuid(?:Apple(?:CSP(?:DL)?|DotMac(?:DL|TP)|FileDL|LDAPDL|SdCSPDL|X509(?:CL|TP))|Cssm)|k(?:A(?:ERemoteProcess(?:NameKey|ProcessIDKey|U(?:RLKey|serIDKey))|X(?:A(?:ttachmentTextAttribute|utocorrectedTextAttribute)|BackgroundColorTextAttribute|Fo(?:nt(?:FamilyKey|NameKey|SizeKey|TextAttribute)|reg(?:oundColorTextAttribute|roundColorTextAttribute))|LinkTextAttribute|MisspelledTextAttribute|NaturalLanguageTextAttribute|ReplacementStringTextAttribute|S(?:hadowTextAttribute|trikethrough(?:ColorTextAttribute|TextAttribute)|uperscriptTextAttribute)|Underline(?:ColorTextAttribute|TextAttribute)|V(?:alue(?:AXErrorType|C(?:FRangeType|G(?:PointType|RectType|SizeType))|IllegalType)|isibleNameKey))|u(?:dioStreamAnyRate|thorizationExternalFormLength))|C(?:F(?:DNSServiceFailureKey|ErrorDomain(?:C(?:FNetwork|GImageMetadata)|SystemConfiguration|WinSock)|FTPStatusCodeKey|GetAddrInfoFailureKey|HTTP(?:Authentication(?:AccountDomain|Password|Scheme(?:Basic|Digest|Kerberos|N(?:TLM|egotiate(?:2)?)|XMobileMeAuthToken)|Username)|Version1_(?:0|1))|NetworkProxies(?:Exc(?:eptionsList|ludeSimpleHostnames)|FTP(?:Enable|P(?:assive|ort|roxy))|Gopher(?:Enable|P(?:ort|roxy))|HTTP(?:Enable|P(?:ort|roxy)|S(?:Enable|P(?:ort|roxy)))|ProxyAuto(?:Config(?:Enable|JavaScript|URLString)|DiscoveryEnable)|RTSP(?:Enable|P(?:ort|roxy))|SOCKS(?:Enable|P(?:ort|roxy)))|Proxy(?:AutoConfiguration(?:HTTPResponseKey|JavaScriptKey|URLKey)|HostNameKey|P(?:asswordKey|ortNumberKey)|Type(?:AutoConfiguration(?:JavaScript|URL)|FTP|HTTP(?:S)?|Key|None|SOCKS)|UsernameKey)|S(?:OCKS(?:NegotiationMethodKey|StatusCodeKey|VersionKey)|tream(?:ErrorDomain(?:FTP|HTTP|Mach|Net(?:DB|Services)|SystemConfiguration|WinSock)|NetworkServiceType(?:Background|V(?:ideo|oice))?|Property(?:ProxyLocalBypass|S(?:SL(?:PeerTrust|Settings)|ocketRemote(?:Host|NetService)))|SSL(?:Certificates|IsServer|Level|PeerName|ValidatesCertificateChain)))|URLErrorFailingURL(?:ErrorKey|StringErrorKey))|GImage(?:Destination(?:BackgroundColor|LossyCompressionQuality)|Property(?:8BIM(?:Dictionary|LayerNames)|C(?:IFF(?:C(?:ameraSerialNumber|ontinuousDrive)|D(?:escription|ictionary)|F(?:irmware|lashExposureComp|ocusMode)|Image(?:FileName|Name|SerialNumber)|LensM(?:axMM|inMM|odel)|Me(?:asuredEV|teringMode)|OwnerName|Re(?:cordID|lease(?:Method|Timing))|S(?:elfTimingTime|hootingMode)|WhiteBalanceIndex)|olorModel(?:CMYK|Gray|Lab|RGB)?)|D(?:NG(?:BackwardVersion|CameraSerialNumber|Dictionary|L(?:ensInfo|ocalizedCameraModel)|UniqueCameraModel|Version)|PI(?:Height|Width)|epth)|Exif(?:A(?:pertureValue|ux(?:Dictionary|F(?:irmware|lashCompensation)|ImageNumber|Lens(?:I(?:D|nfo)|Model|SerialNumber)|OwnerName|SerialNumber))|B(?:odySerialNumber|rightnessValue)|C(?:FAPattern|ameraOwnerName|o(?:lorSpace|mp(?:onentsConfiguration|ressedBitsPerPixel)|ntrast)|ustomRendered)|D(?:ateTime(?:Digitized|Original)|eviceSettingDescription|i(?:ctionary|gitalZoomRatio))|Exposure(?:BiasValue|Index|Mode|Program|Time)|F(?:Number|ileSource|lash(?:Energy|PixVersion)?|ocal(?:Len(?:In35mmFilm|gth)|Plane(?:ResolutionUnit|XResolution|YResolution)))|Ga(?:inControl|mma)|I(?:SOSpeedRatings|mageUniqueID)|L(?:ens(?:M(?:ake|odel)|S(?:erialNumber|pecification))|ightSource)|M(?:a(?:kerNote|xApertureValue)|eteringMode)|OECF|Pixel(?:XDimension|YDimension)|RelatedSoundFile|S(?:aturation|cene(?:CaptureType|Type)|ensingMethod|h(?:arpness|utterSpeedValue)|p(?:atialFrequencyResponse|ectralSensitivity)|ub(?:ject(?:Area|Dist(?:Range|ance)|Location)|secTime(?:Digitized)?))|UserComment|Version|WhiteBalance)|FileSize|G(?:IF(?:D(?:elayTime|ictionary)|HasGlobalColorMap|ImageColorMap|LoopCount|UnclampedDelayTime)|PS(?:A(?:ltitude(?:Ref)?|reaInformation)|D(?:OP|ateStamp|est(?:Bearing(?:Ref)?|Distance(?:Ref)?|L(?:atitude(?:Ref)?|ongitude(?:Ref)?))|i(?:ctionary|fferental))|ImgDirection(?:Ref)?|L(?:atitude(?:Ref)?|ongitude(?:Ref)?)|M(?:apDatum|easureMode)|ProcessingMethod|S(?:atellites|peed(?:Ref)?|tatus)|T(?:imeStamp|rack(?:Ref)?)|Version))|HasAlpha|I(?:PTC(?:ActionAdvised|Byline(?:Title)?|C(?:a(?:ptionAbstract|tegory)|ity|o(?:nt(?:act(?:Info(?:Address|C(?:ity|ountry)|Emails|P(?:hones|ostalCode)|StateProvince|WebURLs))?|entLocation(?:Code|Name))|pyrightNotice|untryPrimaryLocation(?:Code|Name))|re(?:atorContactInfo|dit))|D(?:ateCreated|i(?:ctionary|gitalCreation(?:Date|Time)))|E(?:dit(?:Status|orialUpdate)|xpiration(?:Date|Time))|FixtureIdentifier|Headline|Image(?:Orientation|Type)|Keywords|LanguageIdentifier|O(?:bject(?:AttributeReference|Cycle|Name|TypeReference)|rigina(?:lTransmissionReference|tingProgram))|Pro(?:gramVersion|vinceState)|R(?:e(?:ference(?:Date|Number|Service)|lease(?:Date|Time))|ightsUsageTerms)|S(?:cene|ource|pecialInstructions|tarRating|u(?:b(?:Location|jectReference)|pplementalCategory))|TimeCreated|Urgency|WriterEditor)|s(?:Float|Indexed))|JFIF(?:D(?:ensityUnit|ictionary)|IsProgressive|Version|XDensity|YDensity)|Maker(?:Canon(?:AspectRatioInfo|C(?:ameraSerialNumber|ontinuousDrive)|Dictionary|F(?:irmware|lashExposureComp)|ImageSerialNumber|LensModel|OwnerName)|FujiDictionary|MinoltaDictionary|Nikon(?:C(?:ameraSerialNumber|olorMode)|Di(?:ctionary|gitalZoom)|F(?:lash(?:ExposureComp|Setting)|ocus(?:Distance|Mode))|I(?:SOSe(?:lection|tting)|mageAdjustment)|Lens(?:Adapter|Info|Type)|Quality|Sh(?:arpenMode|ootingMode|utterCount)|WhiteBalanceMode)|OlympusDictionary|PentaxDictionary)|Orientation|P(?:NG(?:Author|C(?:hromaticities|opyright|reationTime)|D(?:escription|ictionary)|Gamma|InterlaceType|ModificationTime|Software|Title|XPixelsPerMeter|YPixelsPerMeter|sRGBIntent)|ixel(?:Height|Width)|rofileName)|RawDictionary|TIFF(?:Artist|Co(?:mpression|pyright)|D(?:ateTime|ictionary|ocumentName)|HostComputer|ImageDescription|M(?:ake|odel)|Orientation|P(?:hotometricInterpretation|rimaryChromaticities)|ResolutionUnit|Software|TransferFunction|WhitePoint|XResolution|YResolution))|Source(?:CreateThumbnail(?:FromImage(?:Always|IfAbsent)|WithTransform)|Should(?:AllowFloat|Cache)|T(?:humbnailMaxPixelSize|ypeIdentifierHint)))|M(?:M(?:ApplyTransformProcName|CreateTransformPropertyProcName|Initialize(?:LinkProfileProcName|TransformProcName))|SEncoderDigestAlgorithmSHA(?:1|256))|SIdentity(?:ErrorDomain|GeneratePosixName)|T(?:F(?:o(?:nt(?:AttributeName|BaselineAdjustAttribute|C(?:ascadeListAttribute|haracterSetAttribute|o(?:llection(?:DisallowAutoActivationOption|IncludeDisabledFontsOption|RemoveDuplicatesOption)|pyrightNameKey))|D(?:es(?:criptionNameKey|igner(?:NameKey|URLNameKey))|isplayNameAttribute)|EnabledAttribute|F(?:amilyName(?:Attribute|Key)|eature(?:Se(?:lector(?:DefaultKey|IdentifierKey|NameKey|SettingKey)|ttingsAttribute)|Type(?:ExclusiveKey|IdentifierKey|NameKey|SelectorsKey)|sAttribute)|ixedAdvanceAttribute|ormatAttribute|ullNameKey)|L(?:anguagesAttribute|icense(?:NameKey|URLNameKey))|Ma(?:cintoshEncodingsAttribute|n(?:ager(?:BundleIdentifier|Error(?:Domain|Font(?:AssetNameKey|DescriptorsKey|URLsKey))|RegisteredFontsChangedNotification)|ufacturerNameKey)|trixAttribute)|NameAttribute|OrientationAttribute|P(?:ostScript(?:CIDNameKey|NameKey)|riorityAttribute)|Registration(?:ScopeAttribute|UserInfoAttribute)|S(?:ampleTextNameKey|izeAttribute|lantTrait|tyleName(?:Attribute|Key)|ubFamilyNameKey|ymbolicTrait)|Tra(?:demarkNameKey|itsAttribute)|U(?:RLAttribute|niqueNameKey)|V(?:ariationA(?:ttribute|xis(?:DefaultValueKey|IdentifierKey|M(?:aximumValueKey|inimumValueKey)|NameKey))|e(?:ndorURLNameKey|rsionNameKey))|W(?:eightTrait|idthTrait))|regroundColor(?:AttributeName|FromContextAttributeName))|rame(?:ClippingPathsAttributeName|P(?:ath(?:ClippingPathAttributeName|FillRuleAttributeName|WidthAttributeName)|rogressionAttributeName)))|GlyphInfoAttributeName|KernAttributeName|LigatureAttributeName|ParagraphStyleAttributeName|RunDelegateAttributeName|S(?:troke(?:ColorAttributeName|WidthAttributeName)|uperscriptAttributeName)|T(?:abColumnTerminatorsAttributeName|ypesetterOptionForcedEmbeddingLevel)|Underline(?:ColorAttributeName|StyleAttributeName)|VerticalFormsAttributeName)|V(?:Buffer(?:MovieTimeKey|NonPropagatedAttachmentsKey|PropagatedAttachmentsKey|Time(?:ScaleKey|ValueKey))|I(?:mageBuffer(?:C(?:GColorSpaceKey|hroma(?:Location(?:BottomFieldKey|TopFieldKey|_(?:Bottom(?:Left)?|Center|DV420|Left|Top(?:Left)?))|Subsampling(?:Key|_4(?:11|2(?:0|2))))|leanAperture(?:H(?:eightKey|orizontalOffsetKey)|Key|VerticalOffsetKey|WidthKey)|olorPrimaries(?:Key|_(?:EBU_3213|ITU_R_709_2|SMPTE_C)))|Display(?:DimensionsKey|HeightKey|WidthKey)|Field(?:CountKey|Detail(?:Key|SpatialFirstLine(?:Early|Late)|Temporal(?:BottomFirst|TopFirst)))|GammaLevelKey|ICCProfileKey|P(?:ixelAspectRatio(?:HorizontalSpacingKey|Key|VerticalSpacingKey)|referredCleanApertureKey)|TransferFunction(?:Key|_(?:ITU_R_709_2|SMPTE_240M_1995|UseGamma))|YCbCrMatrix(?:Key|_(?:ITU_R_(?:601_4|709_2)|SMPTE_240M_1995)))|ndefiniteTime)|Pixel(?:Buffer(?:BytesPerRowAlignmentKey|CG(?:BitmapContextCompatibilityKey|ImageCompatibilityKey)|ExtendedPixels(?:BottomKey|LeftKey|RightKey|TopKey)|HeightKey|IOSurface(?:CoreAnimationCompatibilityKey|OpenGL(?:ES(?:FBOCompatibilityKey|TextureCompatibilityKey)|FBOCompatibilityKey|TextureCompatibilityKey)|PropertiesKey)|MemoryAllocatorKey|OpenGL(?:CompatibilityKey|ES(?:CompatibilityKey|TextureCacheCompatibilityKey))|P(?:ixelFormatTypeKey|laneAlignmentKey|ool(?:AllocationThresholdKey|FreeBufferNotification|M(?:aximumBufferAgeKey|inimumBufferCountKey)))|WidthKey)|Format(?:B(?:itsPerBlock|l(?:ackBlock|ock(?:H(?:eight|orizontalAlignment)|VerticalAlignment|Width)))|C(?:G(?:Bitmap(?:ContextCompatibility|Info)|ImageCompatibility)|o(?:decType|n(?:stant|tainsAlpha)))|F(?:illExtendedPixelsCallback|ourCC)|HorizontalSubsampling|Name|OpenGL(?:Compatibility|ESCompatibility|Format|InternalFormat|Type)|Planes|QDCompatibility|VerticalSubsampling))|ZeroTime)|olorSync(?:A(?:CESCGLinearProfile|dobeRGB1998Profile)|B(?:estQuality|lackPointCompensation)|C(?:ameraDeviceClass|onver(?:sion(?:1DLut|3DLut|BPC|ChannelID|GridPoints|InpChan|Matrix|NDLut|OutChan|ParamCurve(?:0|1|2|3|4))|tQuality)|ustomProfiles)|D(?:CIP3Profile|evice(?:Class|De(?:faultProfileID|scription(?:s)?)|HostScope|ID|ModeDescription(?:s)?|Profile(?:I(?:D|s(?:Current|Default|Factory))|URL|sNotification)|RegisteredNotification|U(?:nregisteredNotification|serScope))|isplay(?:Device(?:Class|ProfilesNotification)|P3Profile)|raftQuality)|F(?:actoryProfiles|ixedPointRange)|Generic(?:CMYKProfile|Gray(?:Gamma22Profile|Profile)|LabProfile|RGBProfile|XYZProfile)|ITUR(?:2020Profile|709Profile)|NormalQuality|Pr(?:eferredCMM|interDeviceClass|ofile(?:C(?:lass|o(?:lorSpace|mputerDomain))|Description|H(?:eader|ostScope)|MD5Digest|PCS|RepositoryChangeNotification|U(?:RL|ser(?:Domain|Scope)))?)|R(?:OMMRGBProfile|e(?:gistrationUpdateWindowServer|nderingIntent(?:Absolute|Perceptual|Relative|Saturation|UseProfileHeader)?))|S(?:RGBProfile|cannerDeviceClass|ig(?:A(?:ToB(?:0Tag|1Tag|2Tag)|bstractClass)|B(?:ToA(?:0Tag|1Tag|2Tag)|lue(?:ColorantTag|TRCTag))|C(?:mykData|o(?:lorSpaceClass|pyrightTag))|D(?:eviceM(?:fgDescTag|odelDescTag)|isplayClass)|G(?:amutTag|r(?:ay(?:Data|TRCTag)|een(?:ColorantTag|TRCTag)))|InputClass|L(?:abData|inkClass)|Media(?:BlackPointTag|WhitePointTag)|NamedColor(?:2Tag|Class)|OutputClass|Pr(?:eview(?:0Tag|1Tag|2Tag)|ofile(?:DescriptionTag|SequenceDescTag))|R(?:ed(?:ColorantTag|TRCTag)|gbData)|TechnologyTag|ViewingCond(?:DescTag|itionsTag)|XYZData))|Transform(?:C(?:odeFragment(?:MD5|Type)|reator)|D(?:eviceTo(?:Device|PCS)|stSpace)|FullConversionData|GamutCheck|Info|P(?:CSTo(?:Device|PCS)|arametricConversionData)|S(?:implifiedConversionData|rcSpace)|Tag)))|D(?:ADiskDescription(?:Bus(?:NameKey|PathKey)|Device(?:GUIDKey|InternalKey|ModelKey|P(?:athKey|rotocolKey)|RevisionKey|TDMLockedKey|UnitKey|VendorKey)|Media(?:B(?:SD(?:M(?:ajorKey|inorKey)|NameKey|UnitKey)|lockSizeKey)|ContentKey|E(?:jectableKey|ncrypt(?:edKey|ionDetailKey))|IconKey|KindKey|LeafKey|NameKey|PathKey|RemovableKey|SizeKey|TypeKey|UUIDKey|W(?:holeKey|ritableKey))|Volume(?:KindKey|MountableKey|N(?:ameKey|etworkKey)|PathKey|TypeKey|UUIDKey))|R(?:A(?:bstractFile|ccessDate|llFilesystems|pplicationIdentifier|ttributeModificationDate|udio(?:FourChannelKey|PreEmphasisKey))|B(?:ackupDate|ibliographicFile|lock(?:Size(?:Key)?|TypeKey)|u(?:fferZone1DataKey|rn(?:AppendableKey|CompletionAction(?:Eject|Key|Mount)|DoubleLayerL0DataZoneBlocksKey|FailureAction(?:Eject|Key|None)|Key|OverwriteDiscKey|RequestedSpeedKey|St(?:atusChangedNotification|rategy(?:BDDAO|CD(?:SAO|TAO)|DVDDAO|IsRequiredKey|Key))|TestingKey|UnderrunProtectionKey|VerifyDiscKey)))|C(?:DText(?:ArrangerKey|C(?:FStringEncodingKey|haracterCodeKey|losedKey|o(?:mposerKey|pyrightAssertedFor(?:NamesKey|SpecialMessagesKey|TitlesKey)))|DiscIdentKey|Genre(?:CodeKey|Key)|Key|LanguageKey|MCNISRCKey|PerformerKey|S(?:izeKey|ongwriterKey|pecialMessageKey)|T(?:OC(?:2Key|Key)|itleKey))|o(?:ntentModificationDate|pyrightFile)|reationDate)|D(?:VD(?:CopyrightInfoKey|TimestampKey)|ata(?:FormKey|Preparer)|e(?:faultDate|vice(?:AppearedNotification|BurnSpeed(?:BD1x|CD1x|DVD1x|HDDVD1x|Max|sKey)|C(?:an(?:TestWrite(?:CDKey|DVDKey)|UnderrunProtect(?:CDKey|DVDKey)|Write(?:BD(?:Key|R(?:EKey|Key))|CD(?:Key|R(?:Key|WKey|awKey)|SAOKey|T(?:AOKey|extKey))|DVD(?:DAOKey|Key|PlusR(?:DoubleLayerKey|Key|W(?:DoubleLayerKey|Key))|R(?:AMKey|DualLayerKey|Key|W(?:DualLayerKey|Key)))|HDDVD(?:Key|R(?:AMKey|DualLayerKey|Key|W(?:DualLayerKey|Key)))|I(?:SRCKey|ndexPointsKey)|Key))|urrentWriteSpeedKey)|DisappearedNotification|FirmwareRevisionKey|I(?:ORegistryEntryPathKey|s(?:BusyKey|TrayOpenKey))|LoadingMechanismCan(?:EjectKey|InjectKey|OpenKey)|M(?:aximumWriteSpeedKey|edia(?:B(?:SDNameKey|locks(?:FreeKey|OverwritableKey|UsedKey))|Class(?:BD|CD|DVD|HDDVD|Key|Unknown)|DoubleLayerL0DataZoneBlocksKey|I(?:nfoKey|s(?:AppendableKey|BlankKey|ErasableKey|OverwritableKey|ReservedKey))|S(?:essionCountKey|tate(?:InTransition|Key|MediaPresent|None))|T(?:rackCountKey|ype(?:BDR(?:E|OM)?|CDR(?:OM|W)?|DVD(?:PlusR(?:DoubleLayer|W(?:DoubleLayer)?)?|R(?:AM|DualLayer|OM|W(?:DualLayer)?)?)|HDDVDR(?:AM|DualLayer|OM|W(?:DualLayer)?)?|Key|Unknown))))|P(?:hysicalInterconnect(?:ATAPI|Fi(?:breChannel|reWire)|Key|Location(?:External|Internal|Key|Unknown)|SCSI|USB)|roductNameKey)|S(?:tatusChangedNotification|upportLevel(?:AppleS(?:hipping|upported)|Key|None|Unsupported|VendorSupported))|Track(?:InfoKey|RefsKey)|VendorNameKey|Write(?:BufferSizeKey|CapabilitiesKey))))|E(?:ffectiveDate|r(?:ase(?:StatusChangedNotification|Type(?:Complete|Key|Quick))|rorStatus(?:AdditionalSenseStringKey|Error(?:InfoStringKey|Key|StringKey)|Key|Sense(?:CodeStringKey|Key)))|xpirationDate)|FreeBlocksKey|HFSPlus(?:CatalogNodeID|TextEncodingHint)?|I(?:SO(?:9660(?:Level(?:One|Two)|VersionNumber)?|Level|MacExtensions|RockRidgeExtensions)|n(?:dexPointsKey|visible))|Joliet|M(?:a(?:c(?:ExtendedFinderFlags|Fi(?:le(?:Creator|Type)|nder(?:Flags|HideExtension))|IconLocation|ScrollPosition|Window(?:Bounds|View))|xBurnSpeedKey)|ediaCatalogNumberKey)|NextWritableAddressKey|P(?:osix(?:FileMode|GID|UID)|reGap(?:IsRequiredKey|LengthKey)|ublisher)|Re(?:cordingDate|fConCFTypeCallbacks)|S(?:CMSCopyright(?:Free|Protected(?:Copy|Original))|e(?:rialCopyManagementStateKey|ssion(?:FormatKey|NumberKey))|tatus(?:Current(?:S(?:essionKey|peedKey)|TrackKey)|EraseTypeKey|P(?:ercentCompleteKey|rogress(?:Current(?:KPS|XFactor)|InfoKey))|State(?:Done|Erasing|F(?:ailed|inishing)|Key|None|Preparing|Session(?:Close|Open)|Track(?:Close|Open|Write)|Verifying)|Total(?:SessionsKey|TracksKey))|u(?:bchannelDataForm(?:Key|None|Pack|Raw)|ppressMacSpecificFiles)|y(?:nchronousBehaviorKey|stemIdentifier))|Track(?:I(?:SRCKey|sEmptyKey)|LengthKey|ModeKey|NumberKey|Packet(?:SizeKey|Type(?:Fixed|Key|Variable))|StartAddressKey|Type(?:Closed|In(?:complete|visible)|Key|Reserved))|UDF(?:ApplicationIdentifierSuffix|ExtendedFilePermissions|InterchangeLevel|Max(?:InterchangeLevel|VolumeSequenceNumber)|PrimaryVolumeDescriptorNumber|RealTimeFile|V(?:ersion1(?:02|50)|olumeSe(?:quenceNumber|t(?:I(?:dentifier|mplementationUse)|Timestamp)))|WriteVersion)?|V(?:erificationType(?:Checksum|Key|None|ProduceAgain|ReceiveData)|olume(?:C(?:heckedDate|reationDate)|E(?:ffectiveDate|xpirationDate)|ModificationDate|Set))))|F(?:CFont(?:CGColorAttribute|Fa(?:ceAttribute|milyAttribute)|NameAttribute|SizeAttribute|VisibleNameAttribute)|ontPanel(?:A(?:TSUFontIDKey|ttribute(?:SizesKey|TagsKey|ValuesKey|sKey))|BackgroundColorAttributeName|Feature(?:SelectorsKey|TypesKey)|MouseTrackingState|Variation(?:AxesKey|ValuesKey)))|HI(?:Delegate(?:AfterKey|BeforeKey)|Object(?:CustomData(?:C(?:DEFProcIDKey|lassIDKey)|DelegateGroupParametersKey|Parameter(?:NamesKey|TypesKey|ValuesKey)|SuperClassIDKey)|InitParam(?:Description|Event(?:Name|Type)|UserName))|T(?:extViewClassID|oolboxVersionNumber)|View(?:MenuContentID|Window(?:C(?:loseBoxID|o(?:llapseBoxID|ntentID))|GrowBoxID|T(?:itleID|oolbar(?:ButtonID|ID))|ZoomBoxID)))|IO(?:MasterPortDefault|Surface(?:AllocSize|BytesPer(?:Element|Row)|CacheMode|Element(?:Height|Width)|Height|Offset|P(?:ixelFormat|lane(?:B(?:ase|ytesPer(?:Element|Row))|Element(?:Height|Width)|Height|Info|Offset|Size|Width))|Width))|JSClassDefinitionEmpty|LSQuarantine(?:Agent(?:BundleIdentifierKey|NameKey)|DataURLKey|OriginURLKey|T(?:imeStampKey|ype(?:CalendarEventAttachment|EmailAttachment|InstantMessageAttachment|Key|Other(?:Attachment|Download)|WebDownload)))|M(?:D(?:Attribute(?:AllValues|DisplayValues|MultiValued|Name|ReadOnlyValues|Type)|ExporterAvaliable|Item(?:A(?:cquisitionM(?:ake|odel)|l(?:bum|titude)|p(?:erture|pl(?:eLoop(?:Descriptors|s(?:KeyFilterType|LoopMode|RootKey))|icationCategories))|ttributeChangeDate|u(?:di(?:ences|o(?:BitRate|ChannelCount|EncodingApplication|SampleRate|TrackNumber))|thor(?:Addresses|EmailAddresses|s)))|BitsPerSample|C(?:FBundleIdentifier|ameraOwner|ity|o(?:decs|lorSpace|m(?:ment|poser)|nt(?:actKeywords|ent(?:CreationDate|ModificationDate|Type(?:Tree)?)|ributors)|pyright|untry|verage)|reator)|D(?:ateAdded|e(?:liveryType|scription)|i(?:rector|splayName)|ownloadedDate|u(?:eDate|rationSeconds))|E(?:XIF(?:GPSVersion|Version)|ditors|mailAddresses|ncodingApplications|x(?:ecutable(?:Architectures|Platform)|posure(?:Mode|Program|TimeS(?:econds|tring))))|F(?:Number|S(?:C(?:ontentChangeDate|reationDate)|HasCustomIcon|I(?:nvisible|s(?:ExtensionHidden|Stationery))|Label|N(?:ame|odeCount)|Owner(?:GroupID|UserID)|Size)|inderComment|lashOnOff|o(?:calLength(?:35mm)?|nts))|G(?:PS(?:AreaInformation|D(?:OP|ateStamp|est(?:Bearing|Distance|L(?:atitude|ongitude))|ifferental)|M(?:apDatum|easureMode)|ProcessingMethod|Status|Track)|enre)|H(?:asAlphaChannel|eadline)|I(?:SOSpeed|dentifier|mageDirection|n(?:formation|st(?:antMessageAddresses|ructions))|s(?:ApplicationManaged|GeneralMIDISequence|LikelyJunk))|K(?:ey(?:Signature|words)|ind)|L(?:a(?:nguages|stUsedDate|titude|yerNames)|ensModel|ongitude|yricist)|M(?:axAperture|e(?:diaTypes|teringMode)|usical(?:Genre|Instrument(?:Category|Name)))|N(?:amedLocation|umberOfPages)|Or(?:ganizations|i(?:entation|ginal(?:Format|Source)))|P(?:a(?:ge(?:Height|Width)|rticipants|th)|erformers|honeNumbers|ixel(?:Count|Height|Width)|ro(?:ducer|fileName|jects)|ublishers)|R(?:e(?:c(?:ipient(?:Addresses|EmailAddresses|s)|ording(?:Date|Year))|dEyeOnOff|solution(?:HeightDPI|WidthDPI))|ights)|S(?:ecurityMethod|peed|t(?:a(?:rRating|teOrProvince)|reamable)|ubject)|T(?:e(?:mpo|xtContent)|heme|i(?:me(?:Signature|stamp)|tle)|otalBitRate)|URL|V(?:ersion|ideoBitRate)|Wh(?:ereFroms|iteBalance))|Label(?:AddedNotification|BundleURL|C(?:hangedNotification|ontentChangeDate)|DisplayName|I(?:con(?:Data|UUID)|sMutuallyExclusiveSetMember)|Kind(?:IsMutuallyExclusiveSetKey|VisibilityKey)?|RemovedNotification|SetsFinderColor|UUID|Visibility)|P(?:rivateVisibility|ublicVisibility)|Query(?:Did(?:FinishNotification|UpdateNotification)|ProgressNotification|ResultContentRelevance|Scope(?:AllIndexed|Computer(?:Indexed)?|Home|Network(?:Indexed)?)|Update(?:AddedItems|ChangedItems|RemovedItems)))|IDI(?:ObjectType_ExternalMask|Property(?:AdvanceScheduleTimeMuSec|C(?:anRoute|onnectionUniqueID)|D(?:eviceID|isplayName|river(?:DeviceEditorApp|Owner|Version))|I(?:mage|s(?:Broadcast|DrumMachine|E(?:ffectUnit|mbeddedEntity)|Mixer|Sampler))|M(?:a(?:nufacturer|x(?:ReceiveChannels|SysExSpeed|TransmitChannels))|odel)|Name|Offline|P(?:anDisruptsStereo|rivate)|Receive(?:Channels|s(?:BankSelect(?:LSB|MSB)|Clock|MTC|Notes|ProgramChanges))|S(?:ingleRealtimeEntity|upports(?:GeneralMIDI|MMC|ShowControl))|Transmit(?:Channels|s(?:BankSelect(?:LSB|MSB)|Clock|MTC|Notes|ProgramChanges))|UniqueID)))|QL(?:PreviewPropertyTextEncodingNameKey|ThumbnailOption(?:IconModeKey|ScaleFactorKey))|S(?:C(?:BondStatusDevice(?:AggregationStatus|Collecting|Distributing)|Comp(?:AnyRegex|Global|HostNames|Interface|Network|S(?:ervice|ystem)|Users)|DynamicStore(?:Domain(?:File|P(?:lugin|refs)|S(?:etup|tate))|Prop(?:Net(?:Interfaces|Primary(?:Interface|Service)|ServiceIDs)|Setup(?:CurrentSet|LastUpdated))|UseSessionKeys)|Ent(?:Net(?:6to4|AirPort|D(?:HCP|NS)|Ethernet|FireWire|I(?:P(?:Sec|v(?:4|6))|nterface)|L(?:2TP|ink)|Modem|P(?:PP(?:Serial|oE)?|roxies)|SMB)|UsersConsoleUser)|Network(?:Interface(?:IPv4|Type(?:6to4|B(?:luetooth|ond)|Ethernet|FireWire|I(?:EEE80211|P(?:Sec|v4)|rDA)|L2TP|Modem|PPP|Serial|VLAN|WWAN))|ProtocolType(?:DNS|IPv(?:4|6)|Proxies|SMB))|Pr(?:ef(?:CurrentSet|NetworkServices|S(?:ets|ystem))|op(?:InterfaceName|MACAddress|Net(?:6to4Relay|DNS(?:DomainName|Options|S(?:e(?:arch(?:Domains|Order)|rver(?:Addresses|Port|Timeout))|ortList|upplementalMatch(?:Domains|Orders)))|EthernetM(?:TU|edia(?:Options|SubType))|I(?:P(?:Sec(?:AuthenticationMethod|ConnectTime|Local(?:Certificate|Identifier(?:Type)?)|RemoteAddress|S(?:haredSecret(?:Encryption)?|tatus)|XAuth(?:Enabled|Name|Password(?:Encryption)?))|v(?:4(?:Addresses|BroadcastAddresses|ConfigMethod|D(?:HCPClientID|estAddresses)|Router|SubnetMasks)|6(?:Addresses|ConfigMethod|DestAddresses|Flags|PrefixLength|Router)))|nterface(?:DeviceName|Hardware|SubType|Type|s))|L(?:2TP(?:IPSecSharedSecret(?:Encryption)?|Transport)|ink(?:Active|Detaching)|ocalHostName)|Modem(?:AccessPointName|Connect(?:Speed|ion(?:Personality|Script))|D(?:ataCompression|evice(?:ContextID|Model|Vendor)|ialMode)|ErrorCorrection|Hold(?:CallWaitingAudibleAlert|DisconnectOnAnswer|Enabled|Reminder(?:Time)?)|Note|PulseDial|Spe(?:aker|ed))|OverridePrimary|P(?:PP(?:A(?:CSPEnabled|uth(?:Name|P(?:assword(?:Encryption)?|ro(?:mpt|tocol))))|C(?:CP(?:Enabled|MPPE(?:128Enabled|40Enabled))|o(?:mm(?:AlternateRemoteAddress|ConnectDelay|DisplayTerminalWindow|Re(?:dial(?:Count|Enabled|Interval)|moteAddress)|TerminalScript|UseTerminalScript)|nnectTime))|D(?:eviceLastCause|i(?:alOnDemand|sconnect(?:On(?:FastUserSwitch|Idle(?:Timer)?|Logout|Sleep)|Time)))|I(?:PCP(?:CompressionVJ|UsePeerDNS)|dleReminder(?:Timer)?)|L(?:CP(?:Compression(?:ACField|PField)|Echo(?:Enabled|Failure|Interval)|M(?:RU|TU)|ReceiveACCM|TransmitACCM)|astCause|ogfile)|OverridePrimary|RetryConnectTime|S(?:essionTimer|tatus)|UseSessionTimer|VerboseLogging)|roxies(?:Exc(?:eptionsList|ludeSimpleHostnames)|FTP(?:Enable|P(?:assive|ort|roxy))|Gopher(?:Enable|P(?:ort|roxy))|HTTP(?:Enable|P(?:ort|roxy)|S(?:Enable|P(?:ort|roxy)))|ProxyAuto(?:Config(?:Enable|JavaScript|URLString)|DiscoveryEnable)|RTSP(?:Enable|P(?:ort|roxy))|SOCKS(?:Enable|P(?:ort|roxy))))|S(?:MB(?:NetBIOSN(?:ame|odeType)|W(?:INSAddresses|orkgroup))|erviceOrder))|SystemComputerName(?:Encoding)?|UserDefinedName|Version))|Resv(?:Inactive|Link)|ValNet(?:I(?:P(?:Sec(?:AuthenticationMethod(?:Certificate|Hybrid|SharedSecret)|LocalIdentifierTypeKeyID|SharedSecretEncryptionKeychain|XAuthPasswordEncryption(?:Keychain|Prompt))|v(?:4ConfigMethod(?:Automatic|BOOTP|DHCP|INFORM|LinkLocal|Manual|PPP)|6ConfigMethod(?:6to4|Automatic|LinkLocal|Manual|RouterAdvertisement)))|nterface(?:SubType(?:L2TP|PPP(?:Serial|oE))|Type(?:6to4|Ethernet|FireWire|IPSec|PPP)))|L2TP(?:IPSecSharedSecretEncryptionKeychain|TransportIP(?:Sec)?)|ModemDialMode(?:IgnoreDialTone|Manual|WaitForDialTone)|PPPAuthP(?:asswordEncryption(?:Keychain|Token)|ro(?:mpt(?:After|Before)|tocol(?:CHAP|EAP|MSCHAP(?:1|2)|PAP)))|SMBNetBIOSNodeType(?:Broadcast|Hybrid|Mixed|Peer)))|K(?:EndTermChars|M(?:aximumTerms|inTermLength)|ProximityIndexing|S(?:t(?:artTermChars|opWords)|ubstitutions)|TermChars)|ec(?:A(?:CLAuthorization(?:Any|De(?:crypt|lete|rive)|E(?:ncrypt|xport(?:Clear|Wrapped))|GenKey|Import(?:Clear|Wrapped)|Keychain(?:Create|Delete|Item(?:Delete|Insert|Modify|Read))|Login|MAC|Sign)|ttr(?:A(?:cc(?:ess|ount)|pplication(?:Label|Tag)|uthenticationType(?:D(?:PA|efault)|HT(?:MLForm|TP(?:Basic|Digest))|MSN|NTLM|RPA)?)|C(?:an(?:De(?:crypt|rive)|Encrypt|Sign|Unwrap|Verify|Wrap)|ertificate(?:Encoding|Type)|omment|reat(?:ionDate|or))|Description|EffectiveKeySize|Generic|Is(?:Extractable|Invisible|Negative|Permanent|Sensitive|suer)|Key(?:Class(?:P(?:rivate|ublic)|Symmetric)?|SizeInBits|Type(?:3DES|AES|CAST|D(?:ES|SA)|ECDSA|R(?:C(?:2|4)|SA))?)|Label|ModificationDate|P(?:RF(?:HmacAlgSHA(?:1|2(?:24|56)|384|512))?|ath|ort|rotocol(?:A(?:FP|ppleTalk)|DAAP|EPPC|FTP(?:Account|Proxy|S)?|HTTP(?:Proxy|S(?:Proxy)?)?|I(?:MAP(?:S)?|PP|RC(?:S)?)|LDAP(?:S)?|NNTP(?:S)?|POP3(?:S)?|RTSP(?:Proxy)?|S(?:M(?:B|TP)|OCKS|SH)|Telnet(?:S)?)?|ublicKeyHash)|Rounds|S(?:alt|e(?:curityDomain|r(?:ialNumber|v(?:er|ice)))|ubject(?:KeyID)?)|Type))|Base(?:32Encoding|64Encoding)|C(?:FError(?:Architecture|GuestAttributes|InfoPlist|Pat(?:h|tern)|Re(?:quirementSyntax|source(?:A(?:dded|ltered)|Missing|S(?:eal|ideband))))|lass(?:Certificate|GenericPassword|I(?:dentity|nternetPassword)|Key)?|o(?:de(?:Attribute(?:Architecture|BundleVersion|Subarchitecture|UniversalFileOffset)|Info(?:C(?:MS|dHashes|ertificates|hangedFiles)|D(?:esignatedRequirement|igestAlgorithm(?:s)?)|Entitlements(?:Dict)?|F(?:lags|ormat)|I(?:dentifier|mplicitDesignatedRequirement)|MainExecutable|P(?:List|latformIdentifier)|R(?:equirement(?:Data|s)|untimeVersion)|S(?:ource|tatus)|T(?:eamIdentifier|ime(?:stamp)?|rust)|Unique))|mpressionRatio))|D(?:ecodeTypeAttribute|igest(?:HMAC(?:KeyAttribute|MD5|SHA(?:1|2))|LengthAttribute|MD(?:2|4|5)|SHA(?:1|2)|TypeAttribute))|Enc(?:ode(?:LineLengthAttribute|TypeAttribute)|rypt(?:Key|ionMode))|GuestAttribute(?:A(?:rchitecture|udit)|Canonical|DynamicCode(?:InfoPlist)?|Hash|MachPort|Pid|Subarchitecture)|I(?:VKey|dentityDomain(?:Default|KerberosKDC)|mport(?:Export(?:Access|Keychain|Passphrase)|Item(?:CertChain|Identity|KeyID|Label|Trust))|nputIs(?:AttributeName|Digest|PlainText|Raw))|KeyAttributeName|LineLength(?:64|76)|M(?:atch(?:CaseInsensitive|DiacriticInsensitive|EmailAddressIfPresent|I(?:ssuers|temList)|Limit(?:All|One)?|Policy|S(?:earchList|ubject(?:Contains|EndsWith|StartsWith|WholeString))|TrustedOnly|ValidOnDate|WidthInsensitive)|ode(?:C(?:BCKey|FBKey)|ECBKey|NoneKey|OFBKey))|OID(?:A(?:DC_CERT_POLICY|PPLE_(?:CERT_POLICY|E(?:KU_(?:CODE_SIGNING(?:_DEV)?|ICHAT_(?:ENCRYPTION|SIGNING)|RESOURCE_SIGNING|SYSTEM_IDENTITY)|XTENSION(?:_(?:A(?:AI_INTERMEDIATE|DC_(?:APPLE_SIGNING|DEV_SIGNING)|PPLE(?:ID_INTERMEDIATE|_SIGNING))|CODE_SIGNING|I(?:NTERMEDIATE_MARKER|TMS_INTERMEDIATE)|WWDR_INTERMEDIATE))?))|uthority(?:InfoAccess|KeyIdentifier))|B(?:asicConstraints|iometricInfo)|C(?:SSMKeyStruct|ert(?:Issuer|ificatePolicies)|lientAuth|o(?:llectiveSt(?:ateProvinceName|reetAddress)|mmonName|untryName)|rl(?:DistributionPoints|Number|Reason))|D(?:OTMAC_CERT_(?:E(?:MAIL_(?:ENCRYPT|SIGN)|XTENSION)|IDENTITY|POLICY)|e(?:ltaCrlIndicator|scription))|E(?:KU_IPSec|mail(?:Address|Protection)|xtended(?:KeyUsage(?:Any)?|UseCodeSigning))|GivenName|HoldInstructionCode|I(?:nvalidityDate|ssu(?:erAltName|ingDistributionPoint(?:s)?))|K(?:ERBv5_PKINIT_KP_(?:CLIENT_AUTH|KDC)|eyUsage)|LocalityName|M(?:S_NTPrincipalName|icrosoftSGC)|N(?:ameConstraints|etscape(?:Cert(?:Sequence|Type)|SGC))|O(?:CSPSigning|rganization(?:Name|alUnitName))|P(?:olicy(?:Constraints|Mappings)|rivateKeyUsagePeriod)|QC_Statements|S(?:er(?:ialNumber|verAuth)|t(?:ateProvinceName|reetAddress)|u(?:bject(?:AltName|DirectoryAttributes|EmailAddress|InfoAccess|KeyIdentifier|Picture|SignatureBitmap)|rname))|Ti(?:meStamping|tle)|UseExemptions|X509V(?:1(?:Certificate(?:IssuerUniqueId|SubjectUniqueId)|IssuerName(?:CStruct|LDAP|Std)?|S(?:erialNumber|ignature(?:Algorithm(?:Parameters|TBS)?|CStruct|Struct)?|ubject(?:Name(?:CStruct|LDAP|Std)?|PublicKey(?:Algorithm(?:Parameters)?|CStruct)?))|V(?:alidityNot(?:After|Before)|ersion))|3(?:Certificate(?:CStruct|Extension(?:C(?:Struct|ritical)|Id|Struct|Type|Value|s(?:CStruct|Struct))|NumberOfExtensions)?|SignedCertificate(?:CStruct)?)))|P(?:adding(?:Key|NoneKey|PKCS(?:1Key|5Key|7Key))|olicy(?:Apple(?:CodeSigning|EAP|I(?:DValidation|Psec)|PKINIT(?:Client|Server)|S(?:MIME|SL)|X509Basic)|Client|KU_(?:CRLSign|D(?:ataEncipherment|ecipherOnly|igitalSignature)|EncipherOnly|Key(?:Agreement|CertSign|Encipherment)|NonRepudiation)|MacAppStoreReceipt|Name|Oid)|roperty(?:Key(?:L(?:abel|ocalizedLabel)|Type|Value)|Type(?:Dat(?:a|e)|Error|S(?:ection|tring|uccess)|Title|URL|Warning)))|R(?:andomDefault|eturn(?:Attributes|Data|PersistentRef|Ref))|S(?:haredPassword|ignatureAttributeName)|Transform(?:A(?:bort(?:AttributeName|OriginatorKey)|ction(?:Attribute(?:Notification|Validation)|CanExecute|ExternalizeExtraData|Finalize|InternalizeExtraData|ProcessData|StartingExecution))|DebugAttributeName|ErrorDomain|InputAttributeName|OutputAttributeName|PreviousErrorKey|TransformName)|Use(?:ItemList|Keychain)|Value(?:Data|PersistentRef|Ref)|ZLibEncoding)|peech(?:Audio(?:GraphProperty|UnitProperty)|C(?:haracterModeProperty|ommand(?:DelimiterProperty|Prefix|Suffix)|urrentVoiceProperty)|Dictionary(?:Abbreviations|Entry(?:Phonemes|Spelling)|LocaleIdentifier|ModificationDate|Pronunciations)|Error(?:C(?:FCallBack|allback(?:CharacterOffset|SpokenString)|ount)|Newest(?:CharacterOffset)?|Oldest(?:CharacterOffset)?|sProperty)|InputModeProperty|Mode(?:Literal|Normal|Phoneme|T(?:ext|une))|N(?:o(?:EndingProsody|SpeechInterrupt)|umberModeProperty)|OutputTo(?:AudioDeviceProperty|ExtAudioFileProperty|FileURLProperty)|P(?:honeme(?:CallBack|Info(?:Example|Hilite(?:End|Start)|Opcode|Symbol)|OptionsProperty|SymbolsProperty)|itch(?:BaseProperty|ModProperty)|reflightThenPause)|R(?:ateProperty|e(?:centSyncProperty|fConProperty|setProperty))|S(?:peechDoneCallBack|tatus(?:NumberOfCharactersLeft|Output(?:Busy|Paused)|P(?:honemeCode|roperty))|yn(?:cCallBack|thesizerInfo(?:Identifier|Manufacturer|Property|Version)))|TextDoneCallBack|Vo(?:ice(?:Creator|ID)|lumeProperty)|WordCFCallBack))|T(?:IS(?:Category(?:InkInputSource|KeyboardInputSource|PaletteInputSource)|Notify(?:EnabledKeyboardInputSourcesChanged|SelectedKeyboardInputSourceChanged)|Property(?:BundleID|I(?:con(?:ImageURL|Ref)|nput(?:ModeID|Source(?:Category|I(?:D|s(?:ASCIICapable|Enable(?:Capable|d)|Select(?:Capable|ed)))|Languages|Type)))|LocalizedName|UnicodeKeyLayoutData)|Type(?:CharacterPalette|Ink|Keyboard(?:InputM(?:ethod(?:ModeEnabled|WithoutModes)|ode)|Layout|Viewer)))|XN(?:Action(?:Align(?:Center|Left|Right)|C(?:hange(?:Color|Font(?:Feature|Variation)?|GlyphVariation|S(?:ize|tyle)|TextPosition)|lear|ountOf(?:AllChanges|StyleChanges|TextChanges)|ut)|Drop|Move|Paste|Typing|UndoLast)|D(?:ataOption(?:CharacterEncodingKey|DocumentTypeKey)|ocumentAttribute(?:AuthorKey|C(?:o(?:m(?:mentKey|panyNameKey)|pyrightKey)|reationTimeKey)|EditorKey|KeywordsKey|ModificationTimeKey|SubjectKey|TitleKey))|MLTEDocumentType|PlainTextDocumentType|QuickTimeDocumentType|RTFDocumentType))|UT(?:ExportedTypeDeclarationsKey|ImportedTypeDeclarationsKey|T(?:agClass(?:FilenameExtension|MIMEType|NSPboardType|OSType)|ype(?:A(?:lias(?:File|Record)|ppl(?:e(?:ICNS|ProtectedMPEG4Audio)|ication(?:Bundle|File)?)|rchive|udio(?:visualContent)?)|B(?:MP|undle)|C(?:Header|PlusPlus(?:Header|Source)|Source|o(?:mpositeContent|n(?:formsToKey|t(?:act|ent))))|D(?:ata(?:base)?|escriptionKey|i(?:rectory|skImage))|Executable|F(?:ileURL|latRTFD|older|ramework)|GIF|HTML|I(?:CO|conFileKey|dentifierKey|mage|nkText|tem)|J(?:PEG(?:2000)?|avaSource)|M(?:P(?:3|EG(?:4(?:Audio)?)?)|essage|o(?:untPoint|vie))|ObjectiveC(?:PlusPlusSource|Source)|P(?:DF|ICT|NG|ackage|lainText)|QuickTime(?:Image|Movie)|R(?:TF(?:D)?|e(?:ferenceURLKey|solvable))|S(?:ourceCode|ymLink)|T(?:IFF|XNTextAndMultimediaData|agSpecificationKey|ext)|U(?:RL|TF(?:16(?:ExternalPlainText|PlainText)|8PlainText))|V(?:Card|ersionKey|ideo|olume)|WebArchive|XML))))|mach_task_self_|oid(?:A(?:d(?:CAIssuer|OCSP)|n(?:sip(?:384r1|521r1)|y(?:ExtendedKeyUsage|Policy))|uthority(?:InfoAccess|KeyIdentifier))|BasicConstraints|C(?:ertificatePolicies|o(?:mmonName|untryName)|rlDistributionPoints)|Description|E(?:cP(?:rime(?:192v1|256v1)|ubKey)|mailAddress|ntrustVersInfo|xtendedKeyUsage(?:C(?:lientAuth|odeSigning)|EmailProtection|IPSec|MicrosoftSGC|NetscapeSGC|OCSPSigning|ServerAuth|TimeStamping)?)|F(?:ee|riendlyName)|Google(?:EmbeddedSignedCertificateTimestamp|OCSPSignedCertificateTimestamp)|I(?:nhibitAnyPolicy|ssuerAltName)|KeyUsage|Local(?:KeyId|ityName)|M(?:SNTPrincipalName|d(?:2(?:Rsa)?|4(?:Rsa)?|5(?:Fee|Rsa)?))|N(?:ameConstraints|etscapeCertType)|Organization(?:Name|alUnitName)|P(?:olicy(?:Constraints|Mappings)|rivateKeyUsagePeriod)|Qt(?:Cps|UNotice)|Rsa|S(?:ha(?:1(?:Dsa(?:CommonOIW|OIW)?|Ecdsa|Fee|Rsa(?:OIW)?)?|2(?:24(?:Ecdsa|Rsa)?|56(?:Ecdsa|Rsa)?)|384(?:Ecdsa|Rsa)?|512(?:Ecdsa|Rsa)?)|tateOrProvinceName|ubject(?:AltName|InfoAccess|KeyIdentifier)))|v(?:m_page_(?:mask|s(?:hift|ize))|printf_stderr_func))\\b",
+ "name": "support.variable.c"
},
{
"match": "\\bkCF(?:Islamic(?:TabularCalendar|UmmAlQuraCalendar)|URL(?:AddedToDirectoryDateKey|DocumentIdentifierKey|GenerationIdentifierKey|QuarantinePropertiesKey))\\b",
@@ -133,6 +349,10 @@
"match": "\\bkCFURL(?:CanonicalPathKey|Volume(?:Is(?:EncryptedKey|RootFileSystemKey)|Supports(?:CompressionKey|ExclusiveRenamingKey|FileCloningKey|SwapRenamingKey)))\\b",
"name": "support.variable.cf.10.12.c"
},
+ {
+ "match": "\\bkCF(?:ErrorLocalizedFailureKey|URLVolume(?:AvailableCapacityFor(?:ImportantUsageKey|OpportunisticUsageKey)|Supports(?:AccessPermissionsKey|ImmutableFilesKey)))\\b",
+ "name": "support.variable.cf.10.13.c"
+ },
{
"match": "\\bkCFURL(?:IsExcludedFromBackupKey|PathKey)\\b",
"name": "support.variable.cf.10.8.c"
@@ -142,7 +362,7 @@
"name": "support.variable.cf.10.9.c"
},
{
- "match": "\\bkCF(?:A(?:bsoluteTimeIntervalSince19(?:04|70)|llocator(?:Default|Malloc(?:Zone)?|Null|SystemDefault|UseContext))|B(?:oolean(?:False|True)|u(?:ddhistCalendar|ndle(?:DevelopmentRegionKey|ExecutableKey|I(?:dentifierKey|nfoDictionaryVersionKey)|LocalizationsKey|NameKey|VersionKey)))|C(?:hineseCalendar|o(?:pyString(?:BagCallBacks|DictionaryKeyCallBacks|SetCallBacks)|reFoundationVersionNumber))|DateFormatter(?:AMSymbol|Calendar(?:Name)?|D(?:efault(?:Date|Format)|oesRelativeDateFormattingKey)|EraSymbols|GregorianStartDate|IsLenient|LongEraSymbols|MonthSymbols|PMSymbol|QuarterSymbols|S(?:hort(?:MonthSymbols|QuarterSymbols|Standalone(?:MonthSymbols|QuarterSymbols|WeekdaySymbols)|WeekdaySymbols)|tandalone(?:MonthSymbols|QuarterSymbols|WeekdaySymbols))|T(?:imeZone|woDigitStartDate)|VeryShort(?:MonthSymbols|Standalone(?:MonthSymbols|WeekdaySymbols)|WeekdaySymbols)|WeekdaySymbols)|Error(?:D(?:escriptionKey|omain(?:Cocoa|Mach|OSStatus|POSIX))|FilePathKey|Localized(?:DescriptionKey|FailureReasonKey|RecoverySuggestionKey)|U(?:RLKey|nderlyingErrorKey))|GregorianCalendar|HebrewCalendar|I(?:SO8601Calendar|ndianCalendar|slamicC(?:alendar|ivilCalendar))|JapaneseCalendar|Locale(?:AlternateQuotation(?:BeginDelimiterKey|EndDelimiterKey)|C(?:alendar(?:Identifier)?|o(?:llat(?:ionIdentifier|orIdentifier)|untryCode)|urren(?:cy(?:Code|Symbol)|tLocaleDidChangeNotification))|DecimalSeparator|ExemplarCharacterSet|GroupingSeparator|Identifier|LanguageCode|MeasurementSystem|Quotation(?:BeginDelimiterKey|EndDelimiterKey)|ScriptCode|UsesMetricSystem|VariantCode)|N(?:otFound|u(?:ll|mber(?:Formatter(?:AlwaysShowDecimalSeparator|Currency(?:Code|DecimalSeparator|GroupingSeparator|Symbol)|De(?:cimalSeparator|faultFormat)|ExponentSymbol|FormatWidth|GroupingS(?:eparator|ize)|I(?:n(?:finitySymbol|ternationalCurrencySymbol)|sLenient)|M(?:ax(?:FractionDigits|IntegerDigits|SignificantDigits)|in(?:FractionDigits|IntegerDigits|SignificantDigits|usSign)|ultiplier)|N(?:aNSymbol|egative(?:Prefix|Suffix))|P(?:adding(?:Character|Position)|er(?:MillSymbol|centSymbol)|lusSign|ositive(?:Prefix|Suffix))|Rounding(?:Increment|Mode)|SecondaryGroupingSize|Use(?:GroupingSeparator|SignificantDigits)|ZeroSymbol)|N(?:aN|egativeInfinity)|PositiveInfinity)))|P(?:ersianCalendar|lugIn(?:DynamicRegist(?:erFunctionKey|rationKey)|FactoriesKey|TypesKey|UnloadFunctionKey)|references(?:Any(?:Application|Host|User)|Current(?:Application|Host|User)))|R(?:epublicOfChinaCalendar|unLoop(?:CommonModes|DefaultMode))|S(?:ocket(?:CommandKey|ErrorKey|NameKey|Re(?:gisterCommand|sultKey|trieveCommand)|ValueKey)|tr(?:eamProperty(?:AppendToFile|DataWritten|FileCurrentOffset|Socket(?:NativeHandle|Remote(?:HostName|PortNumber)))|ing(?:BinaryHeapCallBacks|Transform(?:FullwidthHalfwidth|HiraganaKatakana|Latin(?:Arabic|Cyrillic|Greek|H(?:angul|ebrew|iragana)|Katakana|Thai)|MandarinLatin|Strip(?:CombiningMarks|Diacritics)|To(?:Latin|UnicodeName|XMLHex)))))|T(?:imeZoneSystemTimeZoneDidChangeNotification|ype(?:ArrayCallBacks|BagCallBacks|Dictionary(?:KeyCallBacks|ValueCallBacks)|SetCallBacks))|U(?:RL(?:AttributeModificationDateKey|C(?:ontent(?:AccessDateKey|ModificationDateKey)|reationDateKey)|File(?:AllocatedSizeKey|Protection(?:Complete(?:Un(?:lessOpen|tilFirstUserAuthentication))?|Key|None)|Resource(?:IdentifierKey|Type(?:BlockSpecial|CharacterSpecial|Directory|Key|NamedPipe|Regular|S(?:ocket|ymbolicLink)|Unknown))|S(?:ecurityKey|izeKey))|HasHiddenExtensionKey|Is(?:AliasFileKey|DirectoryKey|ExecutableKey|HiddenKey|MountTriggerKey|PackageKey|Re(?:adableKey|gularFileKey)|Sy(?:mbolicLinkKey|stemImmutableKey)|U(?:biquitousItemKey|serImmutableKey)|VolumeKey|WritableKey)|KeysOfUnsetValuesKey|L(?:abelNumberKey|inkCountKey|ocalized(?:LabelKey|NameKey|TypeDescriptionKey))|NameKey|P(?:arentDirectoryURLKey|referredIOBlockSizeKey)|T(?:otalFile(?:AllocatedSizeKey|SizeKey)|ypeIdentifierKey)|UbiquitousItem(?:HasUnresolvedConflictsKey|Is(?:DownloadingKey|Upload(?:edKey|ingKey)))|Volume(?:AvailableCapacityKey|CreationDateKey|I(?:dentifierKey|s(?:AutomountedKey|BrowsableKey|EjectableKey|InternalKey|JournalingKey|LocalKey|Re(?:adOnlyKey|movableKey)))|Localized(?:FormatDescriptionKey|NameKey)|MaximumFileSizeKey|NameKey|ResourceCountKey|Supports(?:AdvisoryFileLockingKey|Case(?:PreservedNamesKey|SensitiveNamesKey)|ExtendedSecurityKey|HardLinksKey|JournalingKey|PersistentIDsKey|R(?:enamingKey|ootDirectoryDatesKey)|S(?:parseFilesKey|ymbolicLinksKey)|VolumeSizesKey|ZeroRunsKey)|TotalCapacityKey|U(?:RL(?:ForRemountingKey|Key)|UIDStringKey)))|serNotification(?:Al(?:ert(?:HeaderKey|MessageKey)|ternateButtonTitleKey)|CheckBoxTitlesKey|DefaultButtonTitleKey|IconURLKey|LocalizationURLKey|OtherButtonTitleKey|P(?:opUp(?:SelectionKey|TitlesKey)|rogressIndicatorValueKey)|SoundURLKey|TextField(?:TitlesKey|ValuesKey)))|XMLTreeError(?:Description|L(?:ineNumber|ocation)|StatusCode))\\b",
+ "match": "\\bkCF(?:A(?:bsoluteTimeIntervalSince19(?:04|70)|llocator(?:Default|Malloc(?:Zone)?|Null|SystemDefault|UseContext))|B(?:oolean(?:False|True)|u(?:ddhistCalendar|ndle(?:DevelopmentRegionKey|ExecutableKey|I(?:dentifierKey|nfoDictionaryVersionKey)|LocalizationsKey|NameKey|VersionKey)))|C(?:hineseCalendar|o(?:pyString(?:BagCallBacks|DictionaryKeyCallBacks|SetCallBacks)|reFoundationVersionNumber))|DateFormatter(?:AMSymbol|Calendar(?:Name)?|D(?:efault(?:Date|Format)|oesRelativeDateFormattingKey)|EraSymbols|GregorianStartDate|IsLenient|LongEraSymbols|MonthSymbols|PMSymbol|QuarterSymbols|S(?:hort(?:MonthSymbols|QuarterSymbols|Standalone(?:MonthSymbols|QuarterSymbols|WeekdaySymbols)|WeekdaySymbols)|tandalone(?:MonthSymbols|QuarterSymbols|WeekdaySymbols))|T(?:imeZone|woDigitStartDate)|VeryShort(?:MonthSymbols|Standalone(?:MonthSymbols|WeekdaySymbols)|WeekdaySymbols)|WeekdaySymbols)|Error(?:D(?:escriptionKey|omain(?:Cocoa|Mach|OSStatus|POSIX))|FilePathKey|Localized(?:DescriptionKey|FailureReasonKey|RecoverySuggestionKey)|U(?:RLKey|nderlyingErrorKey))|GregorianCalendar|HebrewCalendar|I(?:SO8601Calendar|ndianCalendar|slamicC(?:alendar|ivilCalendar))|JapaneseCalendar|Locale(?:AlternateQuotation(?:BeginDelimiterKey|EndDelimiterKey)|C(?:alendar(?:Identifier)?|o(?:llat(?:ionIdentifier|orIdentifier)|untryCode)|urren(?:cy(?:Code|Symbol)|tLocaleDidChangeNotification))|DecimalSeparator|ExemplarCharacterSet|GroupingSeparator|Identifier|LanguageCode|MeasurementSystem|Quotation(?:BeginDelimiterKey|EndDelimiterKey)|ScriptCode|UsesMetricSystem|VariantCode)|N(?:otFound|u(?:ll|mber(?:Formatter(?:AlwaysShowDecimalSeparator|Currency(?:Code|DecimalSeparator|GroupingSeparator|Symbol)|De(?:cimalSeparator|faultFormat)|ExponentSymbol|FormatWidth|GroupingS(?:eparator|ize)|I(?:n(?:finitySymbol|ternationalCurrencySymbol)|sLenient)|M(?:ax(?:FractionDigits|IntegerDigits|SignificantDigits)|in(?:FractionDigits|IntegerDigits|SignificantDigits|usSign)|ultiplier)|N(?:aNSymbol|egative(?:Prefix|Suffix))|P(?:adding(?:Character|Position)|er(?:MillSymbol|centSymbol)|lusSign|ositive(?:Prefix|Suffix))|Rounding(?:Increment|Mode)|SecondaryGroupingSize|Use(?:GroupingSeparator|SignificantDigits)|ZeroSymbol)|N(?:aN|egativeInfinity)|PositiveInfinity)))|P(?:ersianCalendar|lugIn(?:DynamicRegist(?:erFunctionKey|rationKey)|FactoriesKey|TypesKey|UnloadFunctionKey)|references(?:Any(?:Application|Host|User)|Current(?:Application|Host|User)))|R(?:epublicOfChinaCalendar|unLoop(?:CommonModes|DefaultMode))|S(?:ocket(?:CommandKey|ErrorKey|NameKey|Re(?:gisterCommand|sultKey|trieveCommand)|ValueKey)|tr(?:eam(?:ErrorDomainS(?:OCKS|SL)|Property(?:AppendToFile|DataWritten|FileCurrentOffset|S(?:OCKS(?:P(?:assword|roxy(?:Host|Port)?)|User|Version)|houldCloseNativeSocket|ocket(?:NativeHandle|Remote(?:HostName|PortNumber)|SecurityLevel)))|SocketS(?:OCKSVersion(?:4|5)|ecurityLevel(?:N(?:egotiatedSSL|one)|TLSv1)))|ing(?:BinaryHeapCallBacks|Transform(?:FullwidthHalfwidth|HiraganaKatakana|Latin(?:Arabic|Cyrillic|Greek|H(?:angul|ebrew|iragana)|Katakana|Thai)|MandarinLatin|Strip(?:CombiningMarks|Diacritics)|To(?:Latin|UnicodeName|XMLHex)))))|T(?:imeZoneSystemTimeZoneDidChangeNotification|ype(?:ArrayCallBacks|BagCallBacks|Dictionary(?:KeyCallBacks|ValueCallBacks)|SetCallBacks))|U(?:RL(?:AttributeModificationDateKey|C(?:ontent(?:AccessDateKey|ModificationDateKey)|reationDateKey)|File(?:AllocatedSizeKey|Protection(?:Complete(?:Un(?:lessOpen|tilFirstUserAuthentication))?|Key|None)|Resource(?:IdentifierKey|Type(?:BlockSpecial|CharacterSpecial|Directory|Key|NamedPipe|Regular|S(?:ocket|ymbolicLink)|Unknown))|S(?:ecurityKey|izeKey))|HasHiddenExtensionKey|Is(?:AliasFileKey|DirectoryKey|ExecutableKey|HiddenKey|MountTriggerKey|PackageKey|Re(?:adableKey|gularFileKey)|Sy(?:mbolicLinkKey|stemImmutableKey)|U(?:biquitousItemKey|serImmutableKey)|VolumeKey|WritableKey)|KeysOfUnsetValuesKey|L(?:abelNumberKey|inkCountKey|ocalized(?:LabelKey|NameKey|TypeDescriptionKey))|NameKey|P(?:arentDirectoryURLKey|referredIOBlockSizeKey)|T(?:otalFile(?:AllocatedSizeKey|SizeKey)|ypeIdentifierKey)|UbiquitousItem(?:HasUnresolvedConflictsKey|Is(?:DownloadingKey|Upload(?:edKey|ingKey)))|Volume(?:AvailableCapacityKey|CreationDateKey|I(?:dentifierKey|s(?:AutomountedKey|BrowsableKey|EjectableKey|InternalKey|JournalingKey|LocalKey|Re(?:adOnlyKey|movableKey)))|Localized(?:FormatDescriptionKey|NameKey)|MaximumFileSizeKey|NameKey|ResourceCountKey|Supports(?:AdvisoryFileLockingKey|Case(?:PreservedNamesKey|SensitiveNamesKey)|ExtendedSecurityKey|HardLinksKey|JournalingKey|PersistentIDsKey|R(?:enamingKey|ootDirectoryDatesKey)|S(?:parseFilesKey|ymbolicLinksKey)|VolumeSizesKey|ZeroRunsKey)|TotalCapacityKey|U(?:RL(?:ForRemountingKey|Key)|UIDStringKey)))|serNotification(?:Al(?:ert(?:HeaderKey|MessageKey|TopMostKey)|ternateButtonTitleKey)|CheckBoxTitlesKey|DefaultButtonTitleKey|IconURLKey|KeyboardTypesKey|LocalizationURLKey|OtherButtonTitleKey|P(?:opUp(?:SelectionKey|TitlesKey)|rogressIndicatorValueKey)|SoundURLKey|TextField(?:TitlesKey|ValuesKey)))|XMLTreeError(?:Description|L(?:ineNumber|ocation)|StatusCode))\\b",
"name": "support.variable.cf.c"
},
{
@@ -157,6 +377,22 @@
"match": "\\bkCGColor(?:ConversionBlackPointCompensation|Space(?:Extended(?:Gray|Linear(?:Gray|SRGB)|SRGB)|Linear(?:Gray|SRGB)))\\b",
"name": "support.variable.quartz.10.12.c"
},
+ {
+ "match": "\\bkCG(?:Color(?:ConversionTRCSize|SpaceGenericLab)|PDF(?:ContextAccessPermissions|Outline(?:Children|Destination(?:Rect)?|Title)))\\b",
+ "name": "support.variable.quartz.10.13.c"
+ },
+ {
+ "match": "\\bkCGColorSpace(?:DisplayP3_HLG|ExtendedLinear(?:DisplayP3|ITUR_2020)|ITUR_2020_HLG)\\b",
+ "name": "support.variable.quartz.10.14.c"
+ },
+ {
+ "match": "\\bkCGPDFTagProperty(?:A(?:ctualText|lternativeText)|LanguageText|TitleText)\\b",
+ "name": "support.variable.quartz.10.15.c"
+ },
+ {
+ "match": "\\bkCGColorSpace(?:DisplayP3_PQ|ITUR_2020_PQ)\\b",
+ "name": "support.variable.quartz.10.16.c"
+ },
{
"match": "\\bkCGDisplayS(?:howDuplicateLowResolutionModes|tream(?:ColorSpace|DestinationRect|MinimumFrameTime|PreserveAspectRatio|QueueDepth|S(?:howCursor|ourceRect)|YCbCrMatrix(?:_(?:ITU_R_(?:601_4|709_2)|SMPTE_240M_1995))?))\\b",
"name": "support.variable.quartz.10.8.c"
@@ -169,6 +405,17 @@
"repository": {
"functions": {
"patterns": [
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "invalid.deprecated.10.10.support.function.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:AudioFileReadPackets|LS(?:C(?:anRefAcceptItem|opy(?:ApplicationForMIMEType|DisplayNameForRef|Item(?:Attribute(?:s)?|InfoForRef)|KindStringFor(?:MIMEType|Ref|TypeInfo)))|FindApplicationForInfo|GetApplicationFor(?:I(?:nfo|tem)|URL)|Open(?:Application|F(?:SRef|romRefSpec)|ItemsWithRole|URLsWithRole)|RegisterFSRef|S(?:et(?:ExtensionHiddenForRef|ItemAttribute)|haredFileListI(?:nsertItemFSRef|temResolve)))|launch_(?:data_(?:a(?:lloc|rray_(?:get_(?:count|index)|set_index))|copy|dict_(?:get_count|i(?:nsert|terate)|lookup|remove)|free|get_(?:bool|errno|fd|integer|machport|opaque(?:_size)?|real|string|type)|new_(?:bool|fd|integer|machport|opaque|real|string)|set_(?:bool|fd|integer|machport|opaque|real|string))|get_fd|msg))\\b)"
+ },
{
"captures": {
"1": {
@@ -180,6 +427,17 @@
},
"match": "(\\s*)(\\bCF(?:AbsoluteTime(?:AddGregorianUnits|Get(?:D(?:ayOf(?:Week|Year)|ifferenceAsGregorianUnits)|GregorianDate|WeekOfYear))|GregorianDate(?:GetAbsoluteTime|IsValid)|PropertyList(?:Create(?:From(?:Stream|XMLData)|XMLData)|WriteToStream))\\b)"
},
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "invalid.deprecated.10.11.support.function.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:AudioHardwareService(?:AddPropertyListener|GetPropertyData(?:Size)?|HasProperty|IsPropertySettable|RemovePropertyListener|SetPropertyData)|CF(?:FTPCreateParsedResourceListing|ReadStreamCreate(?:For(?:HTTPRequest|StreamedHTTPRequest)|WithFTPURL)|WriteStreamCreateWithFTPURL)|LS(?:Copy(?:DisplayNameForURL|ItemInfoForURL|KindStringForURL)|Get(?:ExtensionInfo|HandlerOptionsForContentType)|S(?:et(?:ExtensionHiddenForURL|HandlerOptionsForContentType)|haredFileList(?:AddObserver|C(?:opy(?:Property|Snapshot)|reate)|Get(?:SeedValue|TypeID)|I(?:nsertItemURL|tem(?:Copy(?:DisplayName|IconRef|Property|ResolvedURL)|Get(?:ID|TypeID)|Move|Remove|SetProperty))|Remove(?:AllItems|Observer)|Set(?:Authorization|Property))))|SSLSetEncryptionCertificate)\\b)"
+ },
{
"captures": {
"1": {
@@ -202,6 +460,17 @@
},
"match": "(\\s*)(\\bCGDisplayModeCopyPixelEncoding\\b)"
},
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "invalid.deprecated.10.12.support.function.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:OS(?:Atomic(?:A(?:dd(?:32(?:Barrier)?|64(?:Barrier)?)|nd32(?:Barrier|Orig(?:Barrier)?)?)|CompareAndSwap(?:32(?:Barrier)?|64(?:Barrier)?|Int(?:Barrier)?|Long(?:Barrier)?|Ptr(?:Barrier)?)|Decrement(?:32(?:Barrier)?|64(?:Barrier)?)|Increment(?:32(?:Barrier)?|64(?:Barrier)?)|Or32(?:Barrier|Orig(?:Barrier)?)?|TestAnd(?:Clear(?:Barrier)?|Set(?:Barrier)?)|Xor32(?:Barrier|Orig(?:Barrier)?)?)|MemoryBarrier|SpinLock(?:Lock|Try|Unlock))|SecCertificateCopyNormalized(?:IssuerContent|SubjectContent)|os_log_is_(?:debug_enabled|enabled))\\b)"
+ },
{
"captures": {
"1": {
@@ -211,7 +480,106 @@
"name": "invalid.deprecated.10.12.support.function.clib.c"
}
},
- "match": "(\\s*)(\\bsyscall\\b)"
+ "match": "(\\s*)(\\b(?:arc4random_addrandom|syscall)\\b)"
+ },
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "invalid.deprecated.10.13.support.function.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:C(?:FNetDiagnostic(?:C(?:opyNetworkStatusPassively|reateWith(?:Streams|URL))|DiagnoseProblemInteractively|SetName)|MSDecoderSetSearchKeychain)|DisposeSRCallBackUPP|GetIconRefFromFileInfo|InvokeSRCallBackUPP|NewSRCallBackUPP|Re(?:adIconFromFSRef|gisterIconRefFromFSRef)|S(?:R(?:Add(?:LanguageObject|Text)|C(?:ancelRecognition|hangeLanguageObject|loseRecognitionSystem|o(?:ntinueRecognition|untItems))|Draw(?:RecognizedText|Text)|EmptyLanguageObject|Get(?:IndexedItem|LanguageModel|Property|Reference)|Idle|New(?:Language(?:Model|ObjectFrom(?:DataFile|Handle))|P(?:ath|hrase)|Recognizer|Word)|OpenRecognitionSystem|P(?:rocess(?:Begin|End)|utLanguageObjectInto(?:DataFile|Handle))|Re(?:leaseObject|move(?:IndexedItem|LanguageObject))|S(?:et(?:IndexedItem|LanguageModel|Property)|pe(?:ak(?:AndDrawText|Text)|echBusy)|t(?:artListening|op(?:Listening|Speech))))|ec(?:CertificateCopySerialNumber|Keychain(?:CopyAccess|SetAccess)|TrustSetKeychains))|UnregisterIconRef|os_trace_(?:debug_enabled|info_enabled|type_enabled))\\b)"
+ },
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "invalid.deprecated.10.13.support.function.quartz.c"
+ }
+ },
+ "match": "(\\s*)(\\bCGColorSpaceC(?:opyICCProfile|reateWithICCProfile)\\b)"
+ },
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "invalid.deprecated.10.14.support.function.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:CVOpenGL(?:Buffer(?:Attach|Create|Get(?:Attributes|TypeID)|Pool(?:Create(?:OpenGLBuffer)?|Get(?:Attributes|OpenGLBufferAttributes|TypeID)|Re(?:lease|tain))|Re(?:lease|tain))|Texture(?:Cache(?:Create(?:TextureFromImage)?|Flush|GetTypeID|Re(?:lease|tain))|Get(?:CleanTexCoords|Name|T(?:arget|ypeID))|IsFlipped|Re(?:lease|tain)))|DR(?:AudioTrackCreate|F(?:SObjectGetRealFSRef|ileCreateReal|olderCreateReal))|SecCertificateCopyPublicKey)\\b)"
+ },
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "invalid.deprecated.10.15.support.function.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:AcquireIconRef|C(?:C_MD(?:2(?:_(?:Final|Init|Update))?|4(?:_(?:Final|Init|Update))?|5(?:_(?:Final|Init|Update))?)|TFont(?:CreateWithQuickdrawInstance|Manager(?:RegisterFontsForURLs|UnregisterFontsForURLs))|ompositeIconRef)|Get(?:CustomIconsEnabled|IconRef(?:From(?:Component|Folder|IconFamilyPtr|TypeInfo)|Owners)?)|Is(?:DataAvailableInIconRef|IconRefComposite|ValidIconRef)|LSCopy(?:AllHandlersForURLScheme|DefaultHandlerForURLScheme)|OverrideIconRef|Re(?:gisterIconRefFromIconFamily|leaseIconRef|moveIconRefOverride)|S(?:SL(?:AddDistinguishedName|C(?:lose|o(?:ntextGetTypeID|py(?:ALPNProtocols|CertificateAuthorities|DistinguishedNames|PeerTrust|RequestedPeerName(?:Length)?))|reateContext)|Get(?:BufferedReadSize|C(?:lientCertificateState|onnection)|D(?:atagramWriteSize|iffieHellmanParams)|EnabledCiphers|MaxDatagramRecordSize|N(?:egotiated(?:Cipher|ProtocolVersion)|umber(?:EnabledCiphers|SupportedCiphers))|P(?:eer(?:DomainName(?:Length)?|ID)|rotocolVersionM(?:ax|in))|S(?:ession(?:Option|State)|upportedCiphers))|Handshake|Re(?:Handshake|ad)|Set(?:ALPNProtocols|C(?:ertificate(?:Authorities)?|lientSideAuthenticate|onnection)|D(?:atagramHelloCookie|iffieHellmanParams)|E(?:nabledCiphers|rror)|IOFuncs|MaxDatagramRecordSize|OCSPResponse|P(?:eer(?:DomainName|ID)|rotocolVersionM(?:ax|in))|Session(?:Config|Option|TicketsEnabled))|Write)|e(?:cTrust(?:Evaluate(?:Async)?|edApplication(?:C(?:opyData|reateFromPath)|SetData))|tCustomIconsEnabled))|UpdateIconRef|sec_protocol_(?:metadata_get_negotiated_(?:ciphersuite|protocol_version)|options_(?:add_tls_ciphersuite(?:_group)?|set_tls_(?:diffie_hellman_parameters|m(?:ax_version|in_version)))))\\b)"
+ },
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "invalid.deprecated.10.15.support.function.cf.c"
+ }
+ },
+ "match": "(\\s*)(\\bCF(?:Bundle(?:CloseBundleResourceMap|OpenBundleResource(?:Files|Map))|URLCopyParameterString)\\b)"
+ },
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "invalid.deprecated.10.15.support.function.quartz.c"
+ }
+ },
+ "match": "(\\s*)(\\bCGColorSpaceIsHDR\\b)"
+ },
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "invalid.deprecated.10.3.support.function.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:DisposeGetScrapDataUPP|InvokeGetScrapDataUPP|NewGetScrapDataUPP|ReleaseFolder)\\b)"
+ },
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "invalid.deprecated.10.4.support.function.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:AH(?:GotoMainTOC|RegisterHelpBook)|CFNetServiceRe(?:gister|solve)|Dispose(?:CaretHookUPP|DrawHookUPP|EOLHookUPP|Hi(?:ghHookUPP|tTestHookUPP)|NWidthHookUPP|T(?:E(?:ClickLoopUPP|DoTextUPP|FindWordUPP|RecalcUPP)|XNActionKeyMapperUPP|extWidthHookUPP)|URL(?:NotifyUPP|SystemEventUPP)|WidthHookUPP)|In(?:s(?:Time|XTime|tall(?:TimeTask|XTimeTask))|voke(?:CaretHookUPP|DrawHookUPP|EOLHookUPP|Hi(?:ghHookUPP|tTestHookUPP)|NWidthHookUPP|T(?:E(?:ClickLoopUPP|DoTextUPP|FindWordUPP|RecalcUPP)|XNActionKeyMapperUPP|extWidthHookUPP)|URL(?:NotifyUPP|SystemEventUPP)|WidthHookUPP))|LM(?:Get(?:ApFontID|SysFontSize)|Set(?:ApFontID|SysFontFam))|New(?:CaretHookUPP|DrawHookUPP|EOLHookUPP|Hi(?:ghHookUPP|tTestHookUPP)|NWidthHookUPP|T(?:E(?:ClickLoopUPP|DoTextUPP|FindWordUPP|RecalcUPP)|XNActionKeyMapperUPP|extWidthHookUPP)|URL(?:NotifyUPP|SystemEventUPP)|WidthHookUPP)|P(?:L(?:pos|str(?:c(?:at|hr|mp|py)|len|nc(?:at|mp|py)|pbrk|rchr|s(?:pn|tr)))|rimeTime(?:Task)?)|R(?:emoveTimeTask|mvTime)|SKSearch(?:Group(?:C(?:opyIndexes|reate)|GetTypeID)|Results(?:C(?:opyMatchingTerms|reateWith(?:Documents|Query))|Get(?:Count|InfoInRange|TypeID))))\\b)"
+ },
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "invalid.deprecated.10.5.support.function.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:A(?:S(?:GetSourceStyles|SetSourceStyles)|UGraph(?:CountNodeConnections|Get(?:ConnectionInfo|N(?:ode(?:Connections|Info)|umberOfConnections))|NewNode)|udio(?:ConverterFillBuffer|Device(?:AddIOProc|Re(?:ad|moveIOProc))|FileComponent(?:DataIsThisFormat|FileIsThisFormat)))|Dispose(?:AVL(?:CompareItemsUPP|DisposeItemUPP|ItemSizeUPP|WalkUPP)|Drag(?:DrawingUPP|ReceiveHandlerUPP|SendDataUPP|TrackingHandlerUPP)|List(?:ClickLoopUPP|DefUPP|SearchUPP)|Menu(?:ItemDrawingUPP|TitleDrawingUPP)|S(?:crapPromiseKeeperUPP|leepQUPP)|Theme(?:ButtonDrawUPP|EraseUPP|IteratorUPP|TabTitleDrawUPP)|Window(?:PaintUPP|TitleDrawingUPP))|Get(?:NameFromSoundBank|ScriptManagerVariable)|Invoke(?:AVL(?:CompareItemsUPP|DisposeItemUPP|ItemSizeUPP|WalkUPP)|Drag(?:DrawingUPP|ReceiveHandlerUPP|SendDataUPP|TrackingHandlerUPP)|List(?:ClickLoopUPP|DefUPP|SearchUPP)|Menu(?:ItemDrawingUPP|TitleDrawingUPP)|S(?:crapPromiseKeeperUPP|leepQUPP)|Theme(?:ButtonDrawUPP|EraseUPP|IteratorUPP|TabTitleDrawUPP)|Window(?:PaintUPP|TitleDrawingUPP))|Music(?:Device(?:PrepareInstrument|ReleaseInstrument)|Sequence(?:LoadSMF(?:DataWithFlags|WithFlags)|Save(?:MIDIFile|SMFData)))|New(?:AVL(?:CompareItemsUPP|DisposeItemUPP|ItemSizeUPP|WalkUPP)|Drag(?:DrawingUPP|ReceiveHandlerUPP|SendDataUPP|TrackingHandlerUPP)|List(?:ClickLoopUPP|DefUPP|SearchUPP)|Menu(?:ItemDrawingUPP|TitleDrawingUPP)|S(?:crapPromiseKeeperUPP|leepQUPP)|Theme(?:ButtonDrawUPP|EraseUPP|IteratorUPP|TabTitleDrawUPP)|Window(?:PaintUPP|TitleDrawingUPP))|S(?:CNetworkInterfaceRefreshConfiguration|etScriptManagerVariable))\\b)"
},
{
"captures": {
@@ -235,6 +603,17 @@
},
"match": "(\\s*)(\\bCG(?:ContextDrawPDFDocument|PDFDocumentGet(?:ArtBox|BleedBox|CropBox|MediaBox|RotationAngle|TrimBox))\\b)"
},
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "invalid.deprecated.10.6.support.function.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:Audio(?:Device(?:AddPropertyListener|GetProperty(?:Info)?|RemovePropertyListener|SetProperty)|File(?:C(?:omponent(?:Create|Initialize|OpenFile)|reate)|Initialize|Open)|Hardware(?:AddPropertyListener|GetProperty(?:Info)?|RemovePropertyListener|SetProperty)|Stream(?:AddPropertyListener|GetProperty(?:Info)?|RemovePropertyListener|SetProperty))|Button|DisposeKCCallbackUPP|ExtAudioFile(?:CreateNew|Open)|FlushEvents|I(?:nvokeKCCallbackUPP|sCmdChar)|KC(?:Add(?:AppleSharePassword|Callback|GenericPassword|I(?:nternetPassword(?:WithPath)?|tem))|C(?:hangeSettings|o(?:pyItem|untKeychains)|reateKeychain)|DeleteItem|Find(?:AppleSharePassword|FirstItem|GenericPassword|InternetPassword(?:WithPath)?|NextItem)|Get(?:Attribute|D(?:ata|efaultKeychain)|IndKeychain|Keychain(?:ManagerVersion|Name)?|Status)|IsInteractionAllowed|Lock|Make(?:AliasFromKCRef|KCRefFrom(?:Alias|FSRef))|NewItem|Re(?:lease(?:Item|Keychain|Search)|moveCallback)|Set(?:Attribute|D(?:ata|efaultKeychain)|InteractionAllowed)|U(?:nlock|pdateItem))|Munger|New(?:KCCallbackUPP|MusicTrackFrom)|S(?:CNetworkCheckReachabilityBy(?:Address|Name)|ecHost(?:CreateGuest|RemoveGuest|Se(?:lect(?:Guest|edGuest)|t(?:GuestStatus|HostingPort))))|UC(?:CreateTextBreakLocator|DisposeTextBreakLocator|FindTextBreak)|kc(?:add(?:applesharepassword|genericpassword|internetpassword(?:withpath)?)|createkeychain|find(?:applesharepassword|genericpassword|internetpassword(?:withpath)?)|getkeychainname|unlock))\\b)"
+ },
{
"captures": {
"1": {
@@ -246,6 +625,17 @@
},
"match": "(\\s*)(\\bCG(?:ConfigureDisplayMode|Display(?:AvailableModes|BestModeForParameters(?:AndRefreshRate)?|CurrentMode|SwitchToMode)|EnableEventStateCombining|FontCreateWithPlatformFont|InhibitLocalEvents|Post(?:KeyboardEvent|MouseEvent|ScrollWheelEvent)|SetLocalEvents(?:FilterDuringSuppressionState|SuppressionInterval))\\b)"
},
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "invalid.deprecated.10.7.support.function.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:Au(?:dioHardware(?:AddRunLoopSource|RemoveRunLoopSource)|thorization(?:CopyPrivilegedReference|ExecuteWithPrivileges))|C(?:MSEncode(?:rSetEncapsulatedContentType)?|SSM_(?:AC_(?:AuthCompute|PassThrough)|C(?:L_(?:C(?:ert(?:Abort(?:Cache|Query)|C(?:ache|reateTemplate)|DescribeFormat|G(?:et(?:All(?:Fields|TemplateFields)|First(?:CachedFieldValue|FieldValue)|KeyInfo|Next(?:CachedFieldValue|FieldValue))|roup(?:FromVerifiedBundle|ToSignedBundle))|Sign|Verify(?:WithKey)?)|rl(?:A(?:bort(?:Cache|Query)|ddCert)|C(?:ache|reateTemplate)|DescribeFormat|Get(?:All(?:CachedRecordFields|Fields)|First(?:CachedFieldValue|FieldValue)|Next(?:CachedFieldValue|FieldValue))|RemoveCert|S(?:etFields|ign)|Verify(?:WithKey)?))|FreeField(?:Value|s)|IsCertInC(?:achedCrl|rl)|PassThrough)|SP_(?:C(?:hangeLogin(?:Acl|Owner)|reate(?:AsymmetricContext|D(?:eriveKeyContext|igestContext)|KeyGenContext|MacContext|PassThroughContext|RandomGenContext|S(?:ignatureContext|ymmetricContext)))|Get(?:Login(?:Acl|Owner)|OperationalStatistics)|Log(?:in|out)|ObtainPrivateKeyFromPublicKey|PassThrough)|hangeKey(?:Acl|Owner))|D(?:L_(?:Authenticate|C(?:hangeDb(?:Acl|Owner)|reateRelation)|D(?:ata(?:AbortQuery|Delete|Get(?:F(?:irst|romUniqueRecordId)|Next)|Insert|Modify)|b(?:C(?:lose|reate)|Delete|Open)|estroyRelation)|Free(?:NameList|UniqueRecord)|GetDb(?:Acl|Name(?:FromHandle|s)|Owner)|PassThrough)|e(?:cryptData(?:Final|Init(?:P)?|P|Update)?|leteContext(?:Attributes)?|riveKey)|igestData(?:Clone|Final|Init|Update)?)|EncryptData(?:Final|Init(?:P)?|P|Update)?|Free(?:Context|Key)|Ge(?:nerate(?:AlgorithmParams|Key(?:P(?:air(?:P)?)?)?|Mac(?:Final|Init|Update)?|Random)|t(?:APIMemoryFunctions|Context(?:Attribute)?|Key(?:Acl|Owner)|ModuleGUIDFromHandle|Privilege|SubserviceUIDFromHandle|TimeValue))|In(?:it|troduce)|ListAttachedModuleManagers|Module(?:Attach|Detach|Load|Unload)|Query(?:KeySizeInBits|Size)|Retrieve(?:Counter|UniqueId)|S(?:et(?:Context|Privilege)|ignData(?:Final|Init|Update)?)|T(?:P_(?:ApplyCrlToDb|C(?:ert(?:CreateTemplate|G(?:etAllTemplateFields|roup(?:Construct|Prune|ToTupleGroup|Verify))|Re(?:claim(?:Abort|Key)|moveFromCrlTemplate|voke)|Sign)|onfirmCredResult|rl(?:CreateTemplate|Sign|Verify))|Form(?:Request|Submit)|PassThrough|Re(?:ceiveConfirmation|trieveCredResult)|SubmitCredRequest|TupleGroupToCertGroup)|erminate)|U(?:n(?:introduce|wrapKey(?:P)?)|pdateContextAttributes)|Verify(?:D(?:ata(?:Final|Init|Update)?|evice)|Mac(?:Final|Init|Update)?)|WrapKey(?:P)?)|reateThreadPool)|Dispose(?:Debugger(?:DisposeThreadUPP|NewThreadUPP|ThreadSchedulerUPP)|Thread(?:EntryUPP|S(?:chedulerUPP|witchUPP)|TerminationUPP)?)|Get(?:CurrentThread|DefaultThreadStackSize|Thread(?:CurrentTaskRef|State(?:GivenTaskRef)?))|I(?:C(?:Add(?:MapEntry|Profile)|Begin|C(?:ount(?:MapEntries|Pr(?:ef|ofiles))|reateGURLEvent)|Delete(?:MapEntry|Pr(?:ef|ofile))|E(?:ditPreferences|nd)|FindPrefHandle|Get(?:C(?:onfigName|urrentProfile)|DefaultPref|Ind(?:MapEntry|Pr(?:ef|ofile))|MapEntry|P(?:erm|r(?:ef(?:Handle)?|ofileName))|Seed|Version)|LaunchURL|Map(?:Entries(?:Filename|TypeCreator)|Filename|TypeCreator)|ParseURL|S(?:e(?:ndGURLEvent|t(?:CurrentProfile|MapEntry|Pr(?:ef(?:Handle)?|ofileName)))|t(?:art|op)))|nvoke(?:Debugger(?:DisposeThreadUPP|NewThreadUPP|ThreadSchedulerUPP)|Thread(?:EntryUPP|S(?:chedulerUPP|witchUPP)|TerminationUPP))|sMetric)|M(?:DS_(?:In(?:itialize|stall)|Terminate|Uninstall)|P(?:A(?:llocate(?:Aligned|TaskStorageIndex)?|rmTimer)|BlockC(?:lear|opy)|C(?:a(?:ncelTimer|useNotification)|reate(?:CriticalRegion|Event|Notification|Queue|Semaphore|T(?:ask|imer))|urrentTaskID)|D(?:e(?:allocateTaskStorageIndex|l(?:ayUntil|ete(?:CriticalRegion|Event|Notification|Queue|Semaphore|Timer)))|isposeTaskException)|E(?:nterCriticalRegion|x(?:it(?:CriticalRegion)?|tractTaskState))|Free|Get(?:AllocatedBlockSize|Next(?:CpuID|TaskID)|TaskStorageValue)|ModifyNotification(?:Parameters)?|NotifyQueue|Processors(?:Scheduled)?|Re(?:gisterDebugger|moteCall(?:CFM)?)|S(?:et(?:E(?:vent|xceptionHandler)|QueueReserve|T(?:ask(?:St(?:ate|orageValue)|Weight)|imerNotify))|ignalSemaphore)|T(?:askIsPreemptive|erminateTask|hrowException)|UnregisterDebugger|Wait(?:ForEvent|On(?:Queue|Semaphore))|Yield)|usicTrackNewExtendedControlEvent)|New(?:Debugger(?:DisposeThreadUPP|NewThreadUPP|ThreadSchedulerUPP)|Thread(?:EntryUPP|S(?:chedulerUPP|witchUPP)|TerminationUPP)?)|Se(?:c(?:A(?:CL(?:C(?:opySimpleContents|reateFromSimpleContents)|GetAuthorizations|Set(?:Authorizations|SimpleContents))|ccess(?:C(?:opySelectedACLList|reateFromOwnerAndACL)|GetOwnerAndACL))|Certificate(?:C(?:opyPreference|reateFromData)|Get(?:AlgorithmID|CLHandle|Data|Issuer|Subject|Type)|SetPreference)|Identity(?:CopyPreference|Se(?:arch(?:C(?:opyNext|reate)|GetTypeID)|tPreference))|Key(?:CreatePair|Ge(?:nerate|tC(?:S(?:PHandle|SMKey)|redentials))|chain(?:Get(?:CSPHandle|DLDBHandle)|Item(?:Export|Get(?:DLDBHandle|UniqueRecordID)|Import)|Search(?:C(?:opyNext|reateFromAttributes)|GetTypeID)))|Policy(?:Get(?:OID|TPHandle|Value)|Se(?:arch(?:C(?:opyNext|reate)|GetTypeID)|tValue))|Trust(?:Get(?:CssmResult(?:Code)?|Result|TPHandle)|SetParameters))|t(?:DebuggerNotificationProcs|Thread(?:ReadyGivenTaskRef|S(?:cheduler|tate(?:EndCritical)?|witcher)|Terminator)))|Thread(?:BeginCritical|CurrentStackSpace|EndCritical)|YieldTo(?:AnyThread|Thread))\\b)"
+ },
{
"captures": {
"1": {
@@ -257,6 +647,17 @@
},
"match": "(\\s*)(\\bCFURLEnumeratorGetSourceDidChange\\b)"
},
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "invalid.deprecated.10.8.support.function.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:A(?:TS(?:CreateFontQueryRunLoopSource|Font(?:A(?:ctivateFrom(?:FileReference|Memory)|pplyFunction)|Deactivate|F(?:amily(?:ApplyFunction|FindFrom(?:Name|QuickDrawName)|Get(?:Encoding|Generation|Name|QuickDrawName)|Iterator(?:Create|Next|Re(?:lease|set)))|indFrom(?:Container|Name|PostScriptName))|Get(?:AutoActivationSettingForApplication|Container(?:FromFileReference)?|F(?:ileReference|ontFamilyResource)|G(?:eneration|lobalAutoActivationSetting)|HorizontalMetrics|Name|PostScriptName|Table(?:Directory)?|VerticalMetrics)|I(?:sEnabled|terator(?:Create|Next|Re(?:lease|set)))|Notif(?:ication(?:Subscribe|Unsubscribe)|y)|Set(?:AutoActivationSettingForApplication|Enabled|GlobalAutoActivationSetting))|GetGeneration)|bsolute(?:DeltaTo(?:Duration|Nanoseconds)|To(?:Duration|Nanoseconds))|dd(?:A(?:bsoluteToAbsolute|tomic(?:16|8)?)|CollectionItem(?:Hdl)?|DurationToAbsolute|FolderDescriptor|NanosecondsToAbsolute|Resource))|B(?:atteryCount|it(?:And(?:Atomic(?:16|8)?)?|Clr|Not|Or(?:Atomic(?:16|8)?)?|S(?:et|hift)|Tst|Xor(?:Atomic(?:16|8)?)?))|C(?:S(?:Copy(?:MachineName|UserName)|GetComponentsThreadMode|SetComponentsThreadMode)|a(?:llComponent(?:C(?:anDo|lose)|Dispatch|Function(?:WithStorage(?:ProcInfo)?)?|Get(?:MPWorkFunction|PublicResource)|Open|Register|Target|Unregister|Version)|ptureComponent)|hangedResource|lo(?:neCollection|se(?:Component(?:ResFile)?|ResFile))|o(?:llectionTagExists|mpareAndSwap|pyCollection|reEndian(?:FlipData|GetFlipper|InstallFlipper)|unt(?:1(?:Resources|Types)|Co(?:llection(?:Items|Owners|Tags)|mponent(?:Instances|s))|Resources|T(?:aggedCollectionItems|ypes)))|ur(?:ResFile|rentProcessorSpeed))|D(?:e(?:bugAssert|crementAtomic(?:16|8)?|l(?:ay|e(?:gateComponentCall|teGestaltValue))|queue|t(?:achResource(?:File)?|ermineIfPathIsEnclosedByFolder))|ispose(?:Co(?:llection(?:ExceptionUPP|FlattenUPP)?|mponent(?:FunctionUPP|MPWorkFunctionUPP|RoutineUPP))|De(?:bug(?:AssertOutputHandlerUPP|Component(?:CallbackUPP)?)|ferredTaskUPP)|ExceptionHandlerUPP|F(?:NSubscriptionUPP|SVolume(?:EjectUPP|MountUPP|UnmountUPP)|olderManagerNotificationUPP)|GetMissingComponentResourceUPP|Handle|IOCompletionUPP|Ptr|ResErrUPP|S(?:electorFunctionUPP|peech(?:DoneUPP|ErrorUPP|PhonemeUPP|SyncUPP|TextDoneUPP|WordUPP))|TimerUPP)|urationTo(?:Absolute|Nanoseconds))|E(?:mpty(?:Collection|Handle)|nqueue)|F(?:N(?:GetDirectoryForSubscription|Notify(?:All|ByPath)?|Subscribe(?:ByPath)?|Unsubscribe)|S(?:AllocateFork|C(?:a(?:ncelVolumeOperation|talogSearch)|lose(?:Fork|Iterator)|o(?:mpareFSRefs|py(?:AliasInfo|D(?:ADiskForVolume|iskIDForVolume)|Object(?:Async|Sync)|URLForVolume))|reate(?:DirectoryUnicode|F(?:ile(?:AndOpenForkUnicode|Unicode)|ork)|Res(?:File|ourceF(?:ile|ork))|StringFromHFSUniStr|VolumeOperation))|D(?:e(?:lete(?:Fork|Object)|termineIfRefIsEnclosedByFolder)|isposeVolumeOperation)|E(?:jectVolume(?:Async|Sync)|xchangeObjects)|F(?:i(?:le(?:Operation(?:C(?:ancel|opyStatus|reate)|GetTypeID|ScheduleWithRunLoop|UnscheduleFromRunLoop)|Security(?:C(?:opyAccessControlList|reate(?:WithFSPermissionInfo)?)|Get(?:Group(?:UUID)?|Mode|Owner(?:UUID)?|TypeID)|RefCreateCopy|Set(?:AccessControlList|Group(?:UUID)?|Mode|Owner(?:UUID)?)))|ndFolder)|lush(?:Fork|Volume)|ollowFinderAlias)|Get(?:Async(?:EjectStatus|MountStatus|UnmountStatus)|CatalogInfo(?:Bulk)?|DataForkName|Fork(?:CBInfo|Position|Size)|HFSUniStrFromString|ResourceForkName|TemporaryDirectoryForReplaceObject|Volume(?:ForD(?:ADisk|iskID)|Info|MountInfo(?:Size)?|Parms))|I(?:s(?:AliasFile|FSRefValid)|terateForks)|LockRange|M(?:a(?:keFSRefUnicode|tchAliasBulk)|o(?:unt(?:LocalVolume(?:Async|Sync)|ServerVolume(?:Async|Sync))|veObject(?:Async|Sync|ToTrash(?:Async|Sync))?))|NewAlias(?:FromPath|Minimal(?:Unicode)?|Unicode)?|Open(?:Fork|Iterator|OrphanResFile|Res(?:File|ourceFile))|Path(?:CopyObject(?:Async|Sync)|FileOperationCopyStatus|GetTemporaryDirectoryForReplaceObject|M(?:akeRef(?:WithOptions)?|oveObject(?:Async|Sync|ToTrash(?:Async|Sync)))|ReplaceObject)|Re(?:adFork|fMakePath|nameUnicode|placeObject|so(?:lve(?:Alias(?:File(?:WithMountFlags)?|WithMountFlags)?|NodeID)|urceFileAlreadyOpen))|Set(?:CatalogInfo|Fork(?:Position|Size)|VolumeInfo)|U(?:n(?:l(?:inkObject|ockRange)|mountVolume(?:Async|Sync))|pdateAlias)|VolumeMount|WriteFork)|i(?:nd(?:Folder|NextComponent)|x(?:2(?:Frac|Long|X)|ATan2|Div|Mul|R(?:atio|ound)))|latten(?:Collection(?:ToHdl)?|PartialCollection)|rac(?:2(?:Fix|X)|Cos|Div|Mul|S(?:in|qrt)))|Ge(?:stalt|t(?:1(?:Ind(?:Resource|Type)|NamedResource|Resource)|Alias(?:Size(?:FromPtr)?|UserType(?:FromPtr)?)|C(?:PUSpeed|o(?:llection(?:DefaultAttributes|ExceptionProc|Item(?:Hdl|Info)?|RetainCount)|mponent(?:In(?:dString|fo|stance(?:Error|Storage))|ListModSeed|Public(?:IndString|Resource(?:List)?)|Re(?:fcon|source)|TypeModSeed)))|Debug(?:ComponentInfo|OptionInfo)|Folder(?:NameUnicode|Types)|HandleSize|Ind(?:Resource|Type|exedCollection(?:Item(?:Hdl|Info)?|Tag))|Ma(?:cOSStatus(?:CommentString|ErrorString)|xResourceSize)|N(?:amedResource|e(?:wCollection|xt(?:FOND|ResourceFile)))|PtrSize|Res(?:Attrs|FileAttrs|Info|ource(?:SizeOnDisk)?)|SpeechInfo|T(?:aggedCollectionItem(?:Info)?|opResourceFile)))|H(?:ClrRBit|GetState|Lock(?:Hi)?|Set(?:RBit|State)|Unlock|and(?:AndHand|ToHand)|omeResFile)|I(?:dentifyFolder|n(?:crementAtomic(?:16|8)?|s(?:ertResourceFile|tall(?:DebugAssertOutputHandler|ExceptionHandler))|v(?:alidateFolderDescriptorCache|oke(?:Co(?:llection(?:ExceptionUPP|FlattenUPP)|mponent(?:MPWorkFunctionUPP|RoutineUPP))|De(?:bug(?:AssertOutputHandlerUPP|ComponentCallbackUPP)|ferredTaskUPP)|ExceptionHandlerUPP|F(?:NSubscriptionUPP|SVolume(?:EjectUPP|MountUPP|UnmountUPP)|olderManagerNotificationUPP)|GetMissingComponentResourceUPP|IOCompletionUPP|ResErrUPP|S(?:electorFunctionUPP|peech(?:DoneUPP|ErrorUPP|PhonemeUPP|SyncUPP|TextDoneUPP|WordUPP))|TimerUPP)))|s(?:H(?:andleValid|eapValid)|PointerValid))|L(?:M(?:Get(?:BootDrive|IntlSpec|MemErr|Res(?:Err|Load)|SysMap|TmpResLoad)|Set(?:BootDrive|IntlSpec|MemErr|Res(?:Err|Load)|Sys(?:FontSize|Map)|TmpResLoad))|o(?:adResource|cale(?:CountNames|Get(?:IndName|Name)|Operation(?:CountLocales|GetLocales))|ng2Fix))|M(?:PSetTaskType|aximumProcessorSpeed|emError|i(?:croseconds|nimumProcessorSpeed))|N(?:anosecondsTo(?:Absolute|Duration)|ew(?:Co(?:llection(?:ExceptionUPP|FlattenUPP)?|mponent(?:FunctionUPP|MPWorkFunctionUPP|RoutineUPP))|De(?:bug(?:AssertOutputHandlerUPP|Component(?:CallbackUPP)?|Option)|ferredTaskUPP)|E(?:mptyHandle|xceptionHandlerUPP)|F(?:NSubscriptionUPP|SVolume(?:EjectUPP|MountUPP|UnmountUPP)|olderManagerNotificationUPP)|Ge(?:staltValue|tMissingComponentResourceUPP)|Handle(?:Clear)?|IOCompletionUPP|Ptr(?:Clear)?|ResErrUPP|S(?:electorFunctionUPP|peech(?:DoneUPP|ErrorUPP|PhonemeUPP|SyncUPP|TextDoneUPP|WordUPP))|TimerUPP))|Open(?:A(?:Component(?:ResFile)?|DefaultComponent)|Component(?:ResFile)?|DefaultComponent)|P(?:B(?:AllocateFork(?:Async|Sync)|C(?:atalogSearch(?:Async|Sync)|lose(?:Fork(?:Async|Sync)|Iterator(?:Async|Sync))|ompareFSRefs(?:Async|Sync)|reate(?:DirectoryUnicode(?:Async|Sync)|F(?:ile(?:AndOpenForkUnicode(?:Async|Sync)|Unicode(?:Async|Sync))|ork(?:Async|Sync))))|Delete(?:Fork(?:Async|Sync)|Object(?:Async|Sync))|ExchangeObjects(?:Async|Sync)|F(?:S(?:CopyFile(?:Async|Sync)|ResolveNodeID(?:Async|Sync))|lush(?:Fork(?:Async|Sync)|Volume(?:Async|Sync)))|Get(?:CatalogInfo(?:Async|Bulk(?:Async|Sync)|Sync)|Fork(?:CBInfo(?:Async|Sync)|Position(?:Async|Sync)|Size(?:Async|Sync))|VolumeInfo(?:Async|Sync))|IterateForks(?:Async|Sync)|M(?:akeFSRefUnicode(?:Async|Sync)|oveObject(?:Async|Sync))|Open(?:Fork(?:Async|Sync)|Iterator(?:Async|Sync))|Re(?:adFork(?:Async|Sync)|nameUnicode(?:Async|Sync))|Set(?:CatalogInfo(?:Async|Sync)|Fork(?:Position(?:Async|Sync)|Size(?:Async|Sync))|VolumeInfo(?:Async|Sync))|UnlinkObject(?:Async|Sync)|WriteFork(?:Async|Sync)|X(?:LockRange(?:Async|Sync)|UnlockRange(?:Async|Sync)))|tr(?:AndHand|To(?:Hand|XHand))|urgeCollection(?:Tag)?)|Re(?:a(?:d(?:Location|PartialResource)|llocateHandle)|coverHandle|gisterComponent(?:FileRef(?:Entries)?|Resource(?:File)?)?|lease(?:Collection|Resource)|move(?:CollectionItem|FolderDescriptor|IndexedCollectionItem|Resource)|place(?:GestaltValue|IndexedCollectionItem(?:Hdl)?)|s(?:Error|olveComponentAlias)|tainCollection)|S(?:64Compare|SL(?:GetProtocolVersion|SetProtocolVersion)|e(?:cTranformCustomGetAttribute|t(?:AliasUserType(?:WithPtr)?|Co(?:llection(?:DefaultAttributes|ExceptionProc|ItemInfo)|mponent(?:Instance(?:Error|Storage)|Refcon))|De(?:bugOptionValue|faultComponent)|GestaltValue|HandleSize|IndexedCollectionItemInfo|PtrSize|Res(?:Attrs|FileAttrs|Info|Load|Purge|ourceSize)|SpeechInfo))|leepQ(?:Install|Remove)|peak(?:Buffer|String|Text)|ub(?:AbsoluteFromAbsolute|DurationFromAbsolute|NanosecondsFromAbsolute)|ysError)|T(?:askLevel|e(?:mpNewHandle|stAnd(?:Clear|Set)|xtToPhonemes)|ickCount)|U(?:64Compare|n(?:captureComponent|flattenCollection(?:FromHdl)?|ique(?:1ID|ID)|registerComponent|signedFixedMulDiv)|p(?:Time|date(?:ResFile|SystemActivity))|se(?:Dictionary|ResFile))|W(?:S(?:Get(?:CFTypeIDFromWSTypeID|WSTypeIDFromCFType)|Method(?:Invocation(?:Add(?:DeserializationOverride|SerializationOverride)|C(?:opy(?:P(?:arameters|roperty)|Serialization)|reate(?:FromSerialization)?)|GetTypeID|Invoke|S(?:cheduleWithRunLoop|et(?:CallBack|P(?:arameters|roperty)))|UnscheduleFromRunLoop)|ResultIsFault)|ProtocolHandler(?:C(?:opy(?:FaultDocument|Property|Re(?:plyD(?:ictionary|ocument)|questD(?:ictionary|ocument)))|reate)|GetTypeID|Set(?:DeserializationOverride|Property|SerializationOverride)))|ide(?:Add|BitShift|Compare|Divide|Multiply|Negate|S(?:hift|quareRoot|ubtract)|WideDivide)|rite(?:PartialResource|Resource))|X2F(?:ix|rac)|annuity|compound|d(?:ec2(?:f|l|num|s(?:tr)?)|tox80)|getaudit|num(?:2dec|tostring)|r(?:andomx|elation)|s(?:etaudit|tr2dec)|x80tod)\\b)"
+ },
{
"captures": {
"1": {
@@ -290,6 +691,17 @@
},
"match": "(\\s*)(\\bCG(?:Re(?:gisterScreenRefreshCallback|leaseScreenRefreshRects)|Screen(?:RegisterMoveCallback|UnregisterMoveCallback)|UnregisterScreenRefreshCallback|W(?:aitForScreen(?:RefreshRects|UpdateRects)|indowServerCFMachPort))\\b)"
},
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "invalid.deprecated.10.9.support.function.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:AX(?:APIEnabled|MakeProcessTrusted|UIElementPostKeyboardEvent)|CopyProcessName|ExitToShell|Get(?:CurrentProcess|FrontProcess|NextProcess|Process(?:BundleLocation|ForPID|Information|PID))|IsProcessVisible|KillProcess|LaunchApplication|ProcessInformationCopyDictionary|S(?:SL(?:Copy(?:PeerCertificates|TrustedRoots)|DisposeContext|Get(?:Allows(?:AnyRoot|Expired(?:Certs|Roots))|EnableCertVerify|ProtocolVersionEnabled|RsaBlinding)|NewContext|Set(?:Allows(?:AnyRoot|Expired(?:Certs|Roots))|EnableCertVerify|ProtocolVersionEnabled|RsaBlinding|TrustedRoots))|ameProcess|e(?:c(?:ChooseIdentity(?:AsSheet)?|DisplayCertificate(?:Group)?|EditTrust(?:AsSheet)?|Policy(?:CreateWithOID|SetProperties))|tFrontProcess(?:WithOptions)?)|howHideProcess)|WakeUpProcess)\\b)"
+ },
{
"captures": {
"1": {
@@ -334,6 +746,116 @@
},
"match": "(\\s*)(\\bCG(?:C(?:ontextS(?:electFont|how(?:Glyphs(?:AtPoint|WithAdvances)?|Text(?:AtPoint)?))|ursorIs(?:DrawnInFramebuffer|Visible))|Display(?:FadeOperationInProgress|I(?:OServicePort|sCaptured)))\\b)"
},
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "invalid.deprecated.tba.support.function.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:AUGraph(?:Add(?:Node|RenderNotify)|C(?:l(?:earConnections|ose)|o(?:nnectNodeInput|untNodeInteractions))|DisconnectNodeInput|Get(?:CPULoad|In(?:dNode|teractionInfo)|MaxCPULoad|N(?:ode(?:Count|In(?:foSubGraph|teractions))|umberOfInteractions))|I(?:nitialize|s(?:Initialized|NodeSubGraph|Open|Running))|N(?:ewNodeSubGraph|odeInfo)|Open|Remove(?:Node|RenderNotify)|S(?:etNodeInputCallback|t(?:art|op))|U(?:ninitialize|pdate))|DisposeAUGraph|NewAUGraph|QL(?:PreviewRequest(?:C(?:opy(?:ContentUTI|Options|URL)|reate(?:Context|PDFContext))|FlushContext|Get(?:DocumentObject|GeneratorBundle|TypeID)|IsCancelled|Set(?:D(?:ataRepresentation|ocumentObject)|URLRepresentation))|Thumbnail(?:C(?:ancel|opy(?:DocumentURL|Image|Options)|reate)|DispatchAsync|Get(?:ContentRect|MaximumSize|TypeID)|IsCancelled|Request(?:C(?:opy(?:ContentUTI|Options|URL)|reateContext)|FlushContext|Get(?:DocumentObject|GeneratorBundle|MaximumSize|TypeID)|IsCancelled|Set(?:DocumentObject|Image(?:AtURL|WithData)?|ThumbnailWith(?:DataRepresentation|URLRepresentation))))))\\b)"
+ },
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "support.function.10.10.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:C(?:GLGetDeviceFromGLRenderer|MS(?:DecoderCopySignerTimestampWithPolicy|EncoderCopySignerTimestampWithPolicy)|TRubyAnnotation(?:Create(?:Copy)?|Get(?:Alignment|Overhang|SizeFactor|T(?:extForPosition|ypeID))))|JSGlobalContext(?:CopyName|SetName)|LSCopy(?:ApplicationURLsForBundleIdentifier|DefaultApplicationURLFor(?:ContentType|URL))|SecAccessControl(?:CreateWithFlags|GetTypeID)|UTType(?:CopyAllTagsWithClass|IsD(?:eclared|ynamic))|launch_activate_socket|os_re(?:lease|tain)|qos_class_(?:main|self)|simd_inverse)\\b)"
+ },
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "support.function.10.11.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:Audio(?:ComponentInstantiate|ServicesPlay(?:AlertSoundWithCompletion|SystemSoundWithCompletion))|C(?:MSEncoderSetSignerAlgorithm|T(?:LineEnumerateCaretOffsets|RunGetBaseAdvancesAndOrigins))|IORegistryEntryCopy(?:FromPath|Path)|JSValueIs(?:Array|Date)|MIDI(?:ClientCreateWithBlock|DestinationCreateWithBlock|InputPortCreateWithBlock)|connectx|disconnectx|xpc_(?:array_get_(?:array|dictionary)|dictionary_get_(?:array|dictionary)))\\b)"
+ },
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "support.function.10.12.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:CTRubyAnnotationCreateWithAttributes|IOSurface(?:AllowsPixelSizeCasting|SetPurgeable)|JS(?:Object(?:Get(?:ArrayBufferByte(?:Length|sPtr)|TypedArray(?:B(?:uffer|yte(?:Length|Offset|sPtr))|Length))|Make(?:ArrayBufferWithBytesNoCopy|TypedArray(?:With(?:ArrayBuffer(?:AndOffset)?|BytesNoCopy))?))|ValueGetTypedArrayType)|MDItemsCopyAttributes|Sec(?:CertificateCopyNormalized(?:IssuerSequence|SubjectSequence)|Key(?:C(?:opy(?:Attributes|ExternalRepresentation|KeyExchangeResult|PublicKey)|reate(?:DecryptedData|EncryptedData|RandomKey|Signature|WithData))|IsAlgorithmSupported|VerifySignature))|os_(?:log_(?:create|type_enabled)|unfair_lock_(?:assert_(?:not_owner|owner)|lock|trylock|unlock))|xpc_connection_activate)\\b)"
+ },
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "support.function.10.13.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:AudioUnitExtension(?:CopyComponentList|SetComponentList)|C(?:GImage(?:DestinationAddAuxiliaryDataInfo|SourceCopyAuxiliaryDataInfoAtIndex)|TFontManagerCreateFontDescriptorsFromData|V(?:ColorPrimariesGet(?:IntegerCodePointForString|StringForIntegerCodePoint)|TransferFunctionGet(?:IntegerCodePointForString|StringForIntegerCodePoint)|YCbCrMatrixGet(?:IntegerCodePointForString|StringForIntegerCodePoint)))|IOSurfaceGet(?:Bit(?:DepthOfComponentOfPlane|OffsetOfComponentOfPlane)|N(?:ameOfComponentOfPlane|umberOfComponentsOfPlane)|RangeOfComponentOfPlane|Subsampling|TypeOfComponentOfPlane)|SecCertificateCopySerialNumberData)\\b)"
+ },
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "support.function.10.14.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:AEDeterminePermissionToAutomateTarget|C(?:GImageSourceGetPrimaryImageIndex|TFramesetterCreateWithTypesetter)|Sec(?:CertificateCopyKey|Trust(?:EvaluateWithError|SetSignedCertificateTimestamps))|sec_(?:certificate_c(?:opy_ref|reate)|identity_c(?:opy_(?:certificates_ref|ref)|reate(?:_with_certificates)?)|protocol_(?:metadata_(?:access_(?:distinguished_names|ocsp_response|peer_certificate_chain|supported_signature_algorithms)|c(?:hallenge_parameters_are_equal|opy_peer_public_key|reate_secret(?:_with_context)?)|get_(?:early_data_accepted|negotiated_(?:protocol|tls_ciphersuite)|server_name)|peers_are_equal)|options_(?:add_(?:pre_shared_key|tls_application_protocol)|set_(?:challenge_block|key_update_block|local_identity|peer_authentication_required|tls_(?:false_start_enabled|is_fallback_attempt|ocsp_enabled|re(?:negotiation_enabled|sumption_enabled)|s(?:ct_enabled|erver_name)|tickets_enabled)|verify_block)))|trust_c(?:opy_ref|reate)))\\b)"
+ },
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "support.function.10.15.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:C(?:GAnimateImage(?:AtURLWithBlock|DataWithBlock)|T(?:FontManager(?:RegisterFont(?:Descriptors|URLs)|UnregisterFont(?:Descriptors|URLs))|GlyphInfoGetGlyph))|JS(?:Object(?:DeletePropertyForKey|GetPropertyForKey|HasPropertyForKey|MakeDeferredPromise|SetPropertyForKey)|Value(?:IsSymbol|MakeSymbol))|SecTrustEvaluateAsyncWithError|aligned_alloc|sec_(?:identity_access_certificates|protocol_(?:metadata_(?:access_pre_shared_keys|get_negotiated_tls_protocol_version)|options_(?:a(?:ppend_tls_ciphersuite(?:_group)?|re_equal)|get_default_m(?:ax_(?:dtls_protocol_version|tls_protocol_version)|in_(?:dtls_protocol_version|tls_protocol_version))|set_(?:m(?:ax_tls_protocol_version|in_tls_protocol_version)|pre_shared_key_selection_block|tls_pre_shared_key_identity_hint))))|xpc_type_get_name)\\b)"
+ },
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "support.function.10.8.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:A(?:ECompareDesc|udioQueueProcessingTapGetQueueTime)|C(?:GImage(?:Destination(?:AddImageAndMetadata|CopyImageSource)|Metadata(?:C(?:opy(?:StringValueWithPath|Tag(?:MatchingImageProperty|WithPath|s))|reate(?:FromXMPData|Mutable(?:Copy)?|XMPData))|EnumerateTagsUsingBlock|Re(?:gisterNamespaceForPrefix|moveTagWithPath)|Set(?:TagWithPath|Value(?:MatchingImageProperty|WithPath))|Tag(?:C(?:opy(?:Name(?:space)?|Prefix|Qualifiers|Value)|reate)|GetType(?:ID)?))|SourceCopyMetadataAtIndex)|MS(?:DecoderCopySigner(?:SigningTime|Timestamp(?:Certificates)?)|EncoderCopySignerTimestamp)|T(?:Font(?:CopyDefaultCascadeListForLanguages|GetOpticalBoundsForGlyphs|Manager(?:RegisterGraphicsFont|UnregisterGraphicsFont))|LineGetBoundsWithOptions)|VImageBufferCreateColorSpaceFromAttachments|opyInstrumentInfoFromSoundBank))\\b)"
+ },
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "support.function.10.9.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:A(?:XIsProcessTrustedWithOptions|udioHardware(?:CreateAggregateDevice|DestroyAggregateDevice))|C(?:GImageSourceRemoveCacheAtIndex|TFont(?:CreateForStringWithLanguage|Descriptor(?:CreateCopyWith(?:Family|SymbolicTraits)|MatchFontDescriptorsWithProgressHandler)))|FSEventStreamSetExclusionPaths|Sec(?:PolicyCreate(?:Revocation|WithProperties)|Trust(?:Copy(?:Exceptions|Result)|GetNetworkFetchAllowed|Set(?:Exceptions|NetworkFetchAllowed|OCSPResponse)))|xpc_activity_(?:copy_criteria|get_state|register|s(?:et_(?:criteria|state)|hould_defer)|unregister))\\b)"
+ },
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "support.function.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:A(?:E(?:Build(?:AppleEvent|Desc|Parameters)|C(?:allObjectAccessor|heckIsRecord|o(?:erce(?:Desc|Ptr)|untItems)|reate(?:AppleEvent|Desc(?:FromExternalPtr)?|List|RemoteProcessResolver))|D(?:e(?:codeMessage|lete(?:Item|Param))|ispose(?:Desc|RemoteProcessResolver|Token)|uplicateDesc)|FlattenDesc|Get(?:A(?:rray|ttribute(?:Desc|Ptr))|CoercionHandler|DescData(?:Range|Size)?|EventHandler|InteractionAllowed|Nth(?:Desc|Ptr)|ObjectAccessor|Param(?:Desc|Ptr)|RegisteredMachPort|SpecialHandler|TheCurrentEvent)|In(?:itializeDesc|stall(?:CoercionHandler|EventHandler|ObjectAccessor|SpecialHandler)|teractWithUser)|ManagerInfo|ObjectInit|P(?:r(?:intDescToHandle|ocess(?:AppleEvent|Event|Message))|ut(?:A(?:rray|ttribute(?:Desc|Ptr))|Desc|P(?:aram(?:Desc|Ptr)|tr)))|Re(?:mo(?:teProcessResolver(?:GetProcesses|ScheduleWithRunLoop)|ve(?:CoercionHandler|EventHandler|ObjectAccessor|SpecialHandler))|placeDescData|s(?:etTimer|olve|umeTheCurrentEvent))|S(?:e(?:nd(?:Message)?|t(?:InteractionAllowed|ObjectCallbacks|TheCurrentEvent))|izeOf(?:Attribute|FlattenedDesc|NthItem|Param)|tream(?:C(?:lose(?:Desc|List|Record)?|reateEvent)|Op(?:en(?:Desc|Event|KeyDesc|List|Record)?|tionalParam)|SetRecordType|Write(?:AEDesc|D(?:ata|esc)|Key(?:Desc)?))|uspendTheCurrentEvent)|UnflattenDesc)|H(?:GotoPage|LookupAnchor|RegisterHelpBookWithURL|Search)|S(?:CopySourceAttributes|GetSourceStyleNames|Init|SetSourceAttributes)|U(?:EventListener(?:AddEventType|Create(?:WithDispatchQueue)?|Notify|RemoveEventType)|Listener(?:AddParameter|Create(?:WithDispatchQueue)?|Dispose|RemoveParameter)|Parameter(?:FormatValue|ListenerNotify|Set|Value(?:FromLinear|ToLinear)))|X(?:IsProcessTrusted|Observer(?:AddNotification|Create(?:WithInfoCallback)?|Get(?:RunLoopSource|TypeID)|RemoveNotification)|UIElement(?:C(?:opy(?:A(?:ction(?:Description|Names)|ttribute(?:Names|Value(?:s)?))|ElementAtPosition|MultipleAttributeValues|ParameterizedAttribute(?:Names|Value))|reate(?:Application|SystemWide))|Get(?:AttributeValueCount|Pid|TypeID)|IsAttributeSettable|PerformAction|Set(?:AttributeValue|MessagingTimeout))|Value(?:Create|Get(?:Type(?:ID)?|Value)))|cquireFirstMatchingEventInQueue|ddEventTypesToHandler|u(?:dio(?:C(?:hannelLayoutTag_GetNumberOfChannels|o(?:dec(?:AppendInput(?:BufferList|Data)|GetProperty(?:Info)?|Initialize|ProduceOutput(?:BufferList|Packets)|Reset|SetProperty|Uninitialize)|mponent(?:Co(?:py(?:ConfigurationInfo|Name)|unt)|FindNext|Get(?:Description|Version)|Instance(?:CanDo|Dispose|GetComponent|New)|Register|Validate)|nverter(?:Convert(?:Buffer|ComplexBuffer)|Dispose|FillComplexBuffer|GetProperty(?:Info)?|New(?:Specific)?|Reset|SetProperty)))|Device(?:CreateIOProcID(?:WithBlock)?|DestroyIOProcID|Get(?:CurrentTime|NearestStartTime)|St(?:art(?:AtTime)?|op)|TranslateTime)|F(?:ile(?:C(?:lose|o(?:mponent(?:C(?:loseFile|ountUserData|reateURL)|ExtensionIsThisFormat|FileDataIsThisFormat|Get(?:GlobalInfo(?:Size)?|Property(?:Info)?|UserData(?:Size)?)|InitializeWithCallbacks|Op(?:en(?:URL|WithCallbacks)|timize)|Re(?:ad(?:Bytes|Packet(?:Data|s))|moveUserData)|Set(?:Property|UserData)|Write(?:Bytes|Packets))|untUserData)|reateWithURL)|Get(?:GlobalInfo(?:Size)?|Property(?:Info)?|UserData(?:Size)?)|InitializeWithCallbacks|Op(?:en(?:URL|WithCallbacks)|timize)|Re(?:ad(?:Bytes|PacketData)|moveUserData)|S(?:et(?:Property|UserData)|tream(?:Close|GetProperty(?:Info)?|Open|ParseBytes|Se(?:ek|tProperty)))|Write(?:Bytes|Packets))|ormatGetProperty(?:Info)?)|HardwareUnload|O(?:bject(?:AddPropertyListener(?:Block)?|GetPropertyData(?:Size)?|HasProperty|IsPropertySettable|RemovePropertyListener(?:Block)?|S(?:etPropertyData|how))|utputUnitSt(?:art|op))|Queue(?:A(?:ddPropertyListener|llocateBuffer(?:WithPacketDescriptions)?)|CreateTimeline|D(?:evice(?:Get(?:CurrentTime|NearestStartTime)|TranslateTime)|ispose(?:Timeline)?)|EnqueueBuffer(?:WithParameters)?|F(?:lush|reeBuffer)|Get(?:CurrentTime|P(?:arameter|roperty(?:Size)?))|New(?:Input(?:WithDispatchQueue)?|Output(?:WithDispatchQueue)?)|OfflineRender|P(?:ause|r(?:ime|ocessingTap(?:Dispose|GetSourceAudio|New)))|Re(?:movePropertyListener|set)|S(?:et(?:OfflineRenderFormat|P(?:arameter|roperty))|t(?:art|op)))|Services(?:AddSystemSoundCompletion|CreateSystemSoundID|DisposeSystemSoundID|GetProperty(?:Info)?|Play(?:AlertSound|SystemSound)|RemoveSystemSoundCompletion|SetProperty)|Unit(?:Add(?:PropertyListener|RenderNotify)|GetP(?:arameter|roperty(?:Info)?)|Initialize|Process(?:Multiple)?|Re(?:move(?:PropertyListenerWithUserData|RenderNotify)|nder|set)|S(?:cheduleParameters|etP(?:arameter|roperty))|Uninitialize))|thorization(?:C(?:opy(?:Info|Rights(?:Async)?)|reate(?:FromExternalForm)?)|Free(?:ItemSet)?|MakeExternalForm|Right(?:Get|Remove|Set))))|C(?:A(?:C(?:lock(?:A(?:ddListener|rm)|B(?:arBeatTimeToBeats|eatsToBarBeatTime)|Dis(?:arm|pose)|Get(?:CurrentT(?:empo|ime)|P(?:layRate|roperty(?:Info)?)|StartTime)|New|ParseMIDI|RemoveListener|S(?:MPTETimeToSeconds|e(?:condsToSMPTETime|t(?:CurrentT(?:empo|ime)|P(?:layRate|roperty)))|t(?:art|op))|TranslateTime)|urrentMediaTime)|Show(?:File)?|Transform3D(?:Concat|EqualToTransform|GetAffineTransform|I(?:nvert|s(?:Affine|Identity))|Make(?:AffineTransform|Rotation|Scale|Translation)|Rotate|Scale|Translate))|C_SHA(?:1(?:_(?:Final|Init|Update))?|2(?:24(?:_(?:Final|Init|Update))?|56(?:_(?:Final|Init|Update))?)|384(?:_(?:Final|Init|Update))?|512(?:_(?:Final|Init|Update))?)|F(?:H(?:TTP(?:Authentication(?:AppliesToRequest|C(?:opy(?:Domains|Method|Realm)|reateFromResponse)|GetTypeID|IsValid|Requires(?:AccountDomain|OrderedRequests|UserNameAndPassword))|Message(?:A(?:ddAuthentication|pp(?:endBytes|lyCredential(?:Dictionary|s)))|C(?:opy(?:AllHeaderFields|Body|HeaderFieldValue|Re(?:quest(?:Method|URL)|sponseStatusLine)|SerializedMessage|Version)|reate(?:Copy|Empty|Re(?:quest|sponse)))|Get(?:ResponseStatusCode|TypeID)|Is(?:HeaderComplete|Request)|Set(?:Body|HeaderFieldValue)))|ost(?:C(?:ancelInfoResolution|reate(?:Copy|With(?:Address|Name)))|Get(?:Addressing|Names|Reachability|TypeID)|S(?:cheduleWithRunLoop|etClient|tartInfoResolution)|UnscheduleFromRunLoop))|Net(?:Service(?:Browser(?:Create|GetTypeID|Invalidate|S(?:cheduleWithRunLoop|earchFor(?:Domains|Services)|topSearch)|UnscheduleFromRunLoop)|C(?:ancel|reate(?:Copy|DictionaryWithTXTData|TXTDataWithDictionary)?)|Get(?:Addressing|Domain|Name|PortNumber|T(?:XTData|argetHost|ype(?:ID)?))|Monitor(?:Create|GetTypeID|Invalidate|S(?:cheduleWithRunLoop|t(?:art|op))|UnscheduleFromRunLoop)|Re(?:gisterWithOptions|solveWithTimeout)|S(?:cheduleWithRunLoop|et(?:Client|TXTData))|UnscheduleFromRunLoop)|work(?:Copy(?:ProxiesFor(?:AutoConfigurationScript|URL)|SystemProxySettings)|ExecuteProxyAutoConfiguration(?:Script|URL)))|S(?:ocketStreamSOCKSGetError(?:Subdomain)?|treamCreatePairWithSocketTo(?:CFHost|NetService)))|G(?:Display(?:CreateUUIDFromDisplayID|GetDisplayIDFromUUID)|Image(?:Destination(?:AddImage(?:FromSource)?|C(?:opyTypeIdentifiers|reateWith(?:Data(?:Consumer)?|URL))|Finalize|GetTypeID|SetProperties)|MetadataGetTypeID|Source(?:C(?:opy(?:Properties(?:AtIndex)?|TypeIdentifiers)|reate(?:I(?:mageAtIndex|ncremental)|ThumbnailAtIndex|With(?:Data(?:Provider)?|URL)))|Get(?:Count|Status(?:AtIndex)?|Type(?:ID)?)|UpdateData(?:Provider)?))|L(?:C(?:hoosePixelFormat|learDrawable|opyContext|reate(?:Context|PBuffer))|D(?:es(?:cribe(?:P(?:Buffer|ixelFormat)|Renderer)|troy(?:Context|P(?:Buffer|ixelFormat)|RendererInfo))|isable)|E(?:nable|rrorString)|FlushDrawable|Get(?:C(?:ontextRetainCount|urrentContext)|GlobalOption|O(?:ffScreen|ption)|P(?:Buffer(?:RetainCount)?|arameter|ixelFormat(?:RetainCount)?)|ShareGroup|V(?:ersion|irtualScreen))|IsEnabled|LockContext|QueryRendererInfo|Re(?:lease(?:Context|P(?:Buffer|ixelFormat))|tain(?:Context|P(?:Buffer|ixelFormat)))|Set(?:CurrentContext|FullScreen(?:OnDisplay)?|GlobalOption|O(?:ffScreen|ption)|P(?:Buffer|arameter)|VirtualScreen)|TexImage(?:IOSurface2D|PBuffer)|U(?:nlockContext|pdateContext)))|M(?:CalibrateDisplay|Plugin(?:ExamineContext|HandleSelection|PostMenuCleanup)|S(?:Decoder(?:C(?:opy(?:AllCerts|Content|DetachedContent|EncapsulatedContentType|Signer(?:Cert|EmailAddress|Status))|reate)|FinalizeMessage|Get(?:NumSigners|TypeID)|IsContentEncrypted|SetDetachedContent|UpdateMessage)|Encode(?:Content|r(?:Add(?:Recipients|S(?:igne(?:dAttributes|rs)|upportingCerts))|C(?:opy(?:Enc(?:apsulatedContentType|odedContent)|Recipients|S(?:igners|upportingCerts))|reate)|Get(?:CertificateChainMode|HasDetachedContent|TypeID)|Set(?:CertificateChainMode|EncapsulatedContentTypeOID|HasDetachedContent)|UpdateContent))))|S(?:Backup(?:IsItemExcluded|SetItemExcluded)|DiskSpace(?:CancelRecovery|GetRecoveryEstimate|StartRecovery)|Get(?:DefaultIdentityAuthority|LocalIdentityAuthority|ManagedIdentityAuthority)|Identity(?:A(?:dd(?:Alias|Member)|uth(?:enticateUsingPassword|ority(?:CopyLocalizedName|GetTypeID)))|C(?:ommit(?:Asynchronously)?|reate(?:Copy|GroupMembershipQuery|PersistentReference)?)|Delete|Get(?:A(?:liases|uthority)|C(?:ertificate|lass)|EmailAddress|FullName|Image(?:Data(?:Type)?|URL)|Posix(?:ID|Name)|TypeID|UUID)|Is(?:Committing|Enabled|Hidden|MemberOfGroup)|Query(?:C(?:opyResults|reate(?:For(?:CurrentUser|Name|P(?:ersistentReference|osixID)|UUID))?)|Execute(?:Asynchronously)?|GetTypeID|Stop)|Remove(?:Alias|Client|Member)|Set(?:Certificate|EmailAddress|FullName|I(?:mage(?:Data|URL)|sEnabled)|Password)))|T(?:F(?:ont(?:C(?:o(?:llection(?:C(?:opy(?:ExclusionDescriptors|FontAttribute(?:s)?|QueryDescriptors)|reate(?:CopyWithFontDescriptors|FromAvailableFonts|M(?:atchingFontDescriptors(?:ForFamily|SortedWithCallback|WithOptions)?|utableCopy)|WithFontDescriptors))|GetTypeID|Set(?:ExclusionDescriptors|QueryDescriptors))|py(?:A(?:ttribute|vailableTables)|CharacterSet|DisplayName|F(?:amilyName|eature(?:Settings|s)|ontDescriptor|ullName)|GraphicsFont|LocalizedName|Name|PostScriptName|SupportedLanguages|T(?:able|raits)|Variation(?:Axes)?))|reate(?:CopyWith(?:Attributes|Family|SymbolicTraits)|ForString|PathForGlyph|UIFontForLanguage|With(?:FontDescriptor(?:AndOptions)?|GraphicsFont|Name(?:AndOptions)?|PlatformFont)))|D(?:escriptor(?:C(?:opy(?:Attribute(?:s)?|LocalizedAttribute)|reate(?:CopyWith(?:Attributes|Feature|Variation)|MatchingFontDescriptor(?:s)?|With(?:Attributes|NameAndSize)))|GetTypeID)|rawGlyphs)|Get(?:A(?:dvancesForGlyphs|scent)|Bounding(?:Box|RectsForGlyphs)|CapHeight|Descent|Glyph(?:Count|WithName|sForCharacters)|L(?:eading|igatureCaretPositions)|Matrix|PlatformFont|S(?:ize|lantAngle|tringEncoding|ymbolicTraits)|TypeID|Un(?:derline(?:Position|Thickness)|itsPerEm)|VerticalTranslationsForGlyphs|XHeight)|Manager(?:C(?:o(?:mpareFontFamilyNames|py(?:Available(?:Font(?:FamilyNames|URLs)|PostScriptNames)|RegisteredFontDescriptors))|reateFont(?:Descriptor(?:FromData|sFromURL)|RequestRunLoopSource))|EnableFontDescriptors|Get(?:AutoActivationSetting|ScopeForURL)|IsSupportedFont|Re(?:gisterFonts(?:ForURL|WithAssetNames)|questFonts)|SetAutoActivationSetting|UnregisterFontsForURL))|rame(?:Draw|Get(?:FrameAttributes|Line(?:Origins|s)|Path|StringRange|TypeID|VisibleStringRange)|setter(?:Create(?:Frame|WithAttributedString)|GetType(?:ID|setter)|SuggestFrameSizeWithConstraints)))|G(?:etCoreTextVersion|lyphInfo(?:CreateWith(?:CharacterIdentifier|Glyph(?:Name)?)|Get(?:Character(?:Collection|Identifier)|GlyphName|TypeID)))|Line(?:Create(?:JustifiedLine|TruncatedLine|WithAttributedString)|Draw|Get(?:Glyph(?:Count|Runs)|ImageBounds|OffsetForStringIndex|PenOffsetForFlush|String(?:IndexForPosition|Range)|T(?:railingWhitespaceWidth|yp(?:eID|ographicBounds))))|ParagraphStyle(?:Create(?:Copy)?|Get(?:TypeID|ValueForSpecifier))|Run(?:D(?:elegate(?:Create|Get(?:RefCon|TypeID))|raw)|Get(?:A(?:dvances(?:Ptr)?|ttributes)|Glyph(?:Count|s(?:Ptr)?)|ImageBounds|Positions(?:Ptr)?|St(?:atus|ring(?:Indices(?:Ptr)?|Range))|T(?:extMatrix|yp(?:eID|ographicBounds))))|T(?:extTab(?:Create|Get(?:Alignment|Location|Options|TypeID))|ypesetter(?:Create(?:Line(?:WithOffset)?|WithAttributedString(?:AndOptions)?)|GetTypeID|Suggest(?:ClusterBreak(?:WithOffset)?|LineBreak(?:WithOffset)?))))|V(?:Buffer(?:GetAttachment(?:s)?|PropagateAttachments|Re(?:lease|moveA(?:llAttachments|ttachment)|tain)|SetAttachment(?:s)?)|DisplayLink(?:CreateWith(?:ActiveCGDisplays|CGDisplay(?:s)?|OpenGLDisplayMask)|Get(?:ActualOutputVideoRefreshPeriod|Current(?:CGDisplay|Time)|NominalOutputVideoRefreshPeriod|OutputVideoLatency|TypeID)|IsRunning|Re(?:lease|tain)|S(?:et(?:CurrentCGDisplay(?:FromOpenGLContext)?|Output(?:Callback|Handler))|t(?:art|op))|TranslateTime)|Get(?:CurrentHostTime|HostClock(?:Frequency|MinimumTimeDelta))|ImageBuffer(?:Get(?:C(?:leanRect|olorSpace)|DisplaySize|EncodedSize)|IsFlipped)|Pixel(?:Buffer(?:Create(?:ResolvedAttributesDictionary|With(?:Bytes|IOSurface|PlanarBytes))?|FillExtendedPixels|Get(?:B(?:aseAddress(?:OfPlane)?|ytesPerRow(?:OfPlane)?)|DataSize|ExtendedPixels|Height(?:OfPlane)?|IOSurface|P(?:ixelFormatType|laneCount)|TypeID|Width(?:OfPlane)?)|IsPlanar|LockBaseAddress|Pool(?:Create(?:PixelBuffer(?:WithAuxAttributes)?)?|Flush|Get(?:Attributes|PixelBufferAttributes|TypeID)|Re(?:lease|tain))|Re(?:lease|tain)|UnlockBaseAddress)|FormatDescription(?:ArrayCreateWithAllPixelFormatTypes|CreateWithPixelFormatType|RegisterDescriptionWithPixelFormatType)))|allNextEventHandler|h(?:ange(?:TextToUnicodeInfo|UnicodeToTextInfo)|eckEventQueueForUserCancel)|o(?:lorSync(?:C(?:MM(?:C(?:opy(?:CMMIdentifier|LocalizedName)|reate)|Get(?:Bundle|TypeID))|reateCodeFragment)|Device(?:CopyDeviceInfo|SetCustomProfiles)|Iterate(?:DeviceProfiles|Installed(?:CMMs|Profiles))|Profile(?:C(?:o(?:ntainsTag|py(?:D(?:ata|escriptionString)|Header|Tag(?:Signatures)?))|reate(?:D(?:eviceProfile|isplayTransferTablesFromVCGT)|Link|Mutable(?:Copy)?|With(?:DisplayID|Name|URL))?)|EstimateGamma(?:WithDisplayID)?|Get(?:DisplayTransferFormulaFromVCGT|MD5|TypeID|URL)|Install|RemoveTag|Set(?:Header|Tag)|Uninstall|Verify)|RegisterDevice|Transform(?:C(?:o(?:nvert|pyProperty)|reate)|GetTypeID|SetProperty)|UnregisterDevice)|n(?:tinueSpeech|vertFrom(?:PStringToUnicode|TextToUnicode|UnicodeTo(?:PString|ScriptCodeRun|Text(?:Run)?)))|py(?:Event(?:As|CGEvent)?|NameFromSoundBank|PhonemesFromText|S(?:peechProperty|ymbolicHotKeys)|ThemeIdentifier)|unt(?:UnicodeMappings|Voices))|reate(?:CompDescriptor|Event(?:WithCGEvent)?|LogicalDescriptor|O(?:bjSpecifier|ffsetDescriptor)|RangeDescriptor|Text(?:Encoding|ToUnicodeInfo(?:ByEncoding)?)|UnicodeToText(?:Info(?:ByEncoding)?|RunInfo(?:By(?:Encoding|ScriptCode))?)))|D(?:A(?:A(?:ddCallbackToSession|pprovalSession(?:Create|GetTypeID|ScheduleWithRunLoop|UnscheduleFromRunLoop))|CallbackCreate|Disk(?:C(?:opy(?:Description|IOMedia|WholeDisk)|reateFrom(?:BSDName|IOMedia|VolumePath))|Get(?:BSDName|TypeID))|GetCallbackFromSession|RemoveCallbackFromSession(?:WithKey)?|Session(?:Create|GetTypeID|S(?:cheduleWithRunLoop|etDispatchQueue)|UnscheduleFromRunLoop))|CS(?:CopyTextDefinition|GetTermRangeInString)|R(?:AudioTrackCreateWithURL|Burn(?:Abort|C(?:opyStatus|reate)|Get(?:Device|Properties|TypeID)|SetProperties|WriteLayout)|C(?:DTextBlock(?:Create(?:ArrayFromPackList)?|Flatten|Get(?:Properties|T(?:rackDictionaries|ypeID)|Value)|Set(?:Properties|TrackDictionaries|Value))|opy(?:DeviceArray|LocalizedStringFor(?:AdditionalSense|DiscRecordingError|SenseCode|Value)))|Device(?:Acquire(?:ExclusiveAccess|MediaReservation)|C(?:loseTray|opy(?:DeviceFor(?:BSDName|IORegistryEntryPath)|Info|Status))|EjectMedia|GetTypeID|IsValid|KPSForXFactor|OpenTray|Release(?:ExclusiveAccess|MediaReservation)|XFactorForKPS)|Erase(?:C(?:opyStatus|reate)|Get(?:Device|Properties|TypeID)|S(?:etProperties|tart))|F(?:SObject(?:Copy(?:BaseName|FilesystemPropert(?:ies|y)|MangledName(?:s)?|RealURL|SpecificName(?:s)?)|Get(?:FilesystemMask|Parent)|IsVirtual|Set(?:BaseName|Filesystem(?:Mask|Propert(?:ies|y))|SpecificName(?:s)?))|ile(?:Create(?:RealWithURL|Virtual(?:Link|With(?:Callback|Data)))|GetTypeID|systemTrack(?:Create|EstimateOverhead))|older(?:AddChild|C(?:o(?:nvertRealToVirtual|pyChildren|untChildren)|reate(?:RealWithURL|Virtual))|GetTypeID|RemoveChild))|Get(?:RefCon|Version)|NotificationCenter(?:AddObserver|Create(?:RunLoopSource)?|GetTypeID|RemoveObserver)|SetRefCon|Track(?:Create|EstimateLength|Get(?:Properties|TypeID)|S(?:etProperties|peedTest)))|ebugPrint(?:Event|MainEventQueue)|is(?:ableSecureEventInput|pose(?:AE(?:Coerce(?:DescUPP|PtrUPP)|DisposeExternalUPP|EventHandlerUPP|FilterUPP|IdleUPP)|C(?:a(?:librate(?:EventUPP|UPP)|nCalibrateUPP)|ontrol(?:ActionUPP|EditTextValidationUPP|KeyFilterUPP|UserPane(?:ActivateUPP|DrawUPP|FocusUPP|HitTestUPP|IdleUPP|KeyDownUPP|TrackingUPP)))|D(?:ataBrowser(?:A(?:cceptDragUPP|ddDragItemUPP)|DrawItemUPP|EditItemUPP|GetContextualMenuUPP|HitTestUPP|Item(?:AcceptDragUPP|CompareUPP|D(?:ataUPP|ragRgnUPP)|HelpContentUPP|Notification(?:UPP|WithItemUPP)|ReceiveDragUPP|UPP)|PostProcessDragUPP|ReceiveDragUPP|SelectContextualMenuUPP|TrackingUPP)|ragInputUPP)|E(?:ditUnicodePostUpdateUPP|vent(?:ComparatorUPP|HandlerUPP|Loop(?:IdleTimerUPP|TimerUPP)))|HM(?:ControlContentUPP|Menu(?:ItemContentUPP|TitleContentUPP)|WindowContentUPP)|I(?:con(?:ActionUPP|GetterUPP)|ndexToUCStringUPP)|M(?:odalFilter(?:UPP|YDUPP)|usic(?:EventIterator|Player|Sequence))|N(?:ColorChangedUPP|MUPP)|OS(?:A(?:ActiveUPP|CreateAppleEventUPP|SendUPP)|L(?:A(?:ccessorUPP|djustMarksUPP)|Co(?:mpareUPP|untUPP)|DisposeTokenUPP|Get(?:ErrDescUPP|MarkTokenUPP)|MarkUPP))|SpeechChannel|T(?:XN(?:ActionNameMapperUPP|ContextualMenuSetupUPP|FindUPP|ScrollInfoUPP)|extToUnicodeInfo)|U(?:nicodeToText(?:FallbackUPP|Info|RunInfo)|serItemUPP))))|E(?:nableSecureEventInput|xtAudioFile(?:CreateWithURL|Dispose|GetProperty(?:Info)?|OpenURL|Read|Se(?:ek|tProperty)|Tell|Wr(?:apAudioFileID|ite(?:Async)?)))|F(?:C(?:Add(?:Collection|FontDescriptorToCollection)|Copy(?:CollectionNames|FontDescriptorsInCollection)|FontDescriptorCreateWith(?:FontAttributes|Name)|Remove(?:Collection|FontDescriptorFromCollection))|P(?:IsFontPanelVisible|ShowHideFontPanel)|SEvent(?:Stream(?:C(?:opy(?:Description|PathsBeingWatched)|reate(?:RelativeToDevice)?)|Flush(?:Async|Sync)|Get(?:DeviceBeingWatched|LatestEventId)|Invalidate|Re(?:lease|tain)|S(?:cheduleWithRunLoop|etDispatchQueue|how|t(?:art|op))|UnscheduleFromRunLoop)|s(?:CopyUUIDForDevice|Get(?:CurrentEventId|LastEventIdForDeviceBeforeTime)|PurgeEventsForDeviceUpToEventId))|indSpecificEventInQueue|lush(?:Event(?:Queue|sMatchingListFromQueue)|SpecificEventsFromQueue))|Get(?:A(?:pplication(?:EventTarget|TextEncoding)|udioUnitParameterDisplayType)|C(?:FRunLoopFromEventLoop|olor|urrent(?:ButtonState|Event(?:ButtonState|KeyModifiers|Loop|Queue|Time)?|KeyModifiers))|Event(?:Class|DispatcherTarget|Kind|MonitorTarget|Parameter|RetainCount|Time)|I(?:con(?:FamilyData|RefVariant)|ndVoice)|Keys|M(?:ainEvent(?:Loop|Queue)|enuTrackingData)|NumEventsInQueue|S(?:criptInfoFromTextEncoding|peech(?:Pitch|Rate)|y(?:mbolicHotKeyMode|stemUIMode))|T(?:extEncoding(?:Base|F(?:ormat|romScriptInfo)|Name|Variant)|hemeMe(?:nu(?:ItemExtra|SeparatorHeight|TitleExtra)|tric))|Voice(?:Description|Info))|HI(?:DictionaryWindowShow|GetMousePosition|MouseTrackingGetParameters|Object(?:AddDelegate|C(?:opy(?:ClassID|Delegates)|reate(?:FromBundle)?)|DynamicCast|FromEventTarget|GetEvent(?:HandlerObject|Target)|Is(?:ArchivingIgnored|OfClass)|PrintDebugInfo|Re(?:gisterSubclass|moveDelegate)|UnregisterClass)|PointConvert|RectConvert|S(?:earchWindowShow|hape(?:C(?:ontainsPoint|reate(?:Copy|Difference|Empty|Intersection|Mutable(?:Copy|WithRect)?|Union|With(?:QDRgn|Rect)|Xor))|Difference|Enumerate|Get(?:AsQDRgn|Bounds|TypeID)|I(?:n(?:set|tersect(?:sRect)?)|s(?:Empty|Rectangular))|Offset|ReplacePathInCGContext|Set(?:Empty|WithShape)|Union(?:WithRect)?|Xor)|izeConvert)|Theme(?:ApplyBackground|B(?:eginFocus|rushCreateCGColor)|Draw(?:B(?:ackground|utton)|ChasingArrows|F(?:ocusRect|rame)|G(?:enericWell|r(?:abber|o(?:upBox|wBox)))|Header|Menu(?:Ba(?:ckground|rBackground)|Item|Separator|Title)|P(?:aneSplitter|lacard|opupArrow)|S(?:crollBarDelimiters|e(?:gment|parator))|T(?:ab(?:Pane)?|extBox|i(?:ckMark|tleBarWidget)|rack(?:TickMarks)?)|WindowFrame)|EndFocus|Get(?:Button(?:BackgroundBounds|ContentBounds|Shape)|GrowBoxBounds|MenuBackgroundShape|ScrollBarTrackRect|T(?:ab(?:DrawShape|Pane(?:ContentShape|DrawShape)|Shape)|ext(?:ColorForThemeBrush|Dimensions)|rack(?:Bounds|DragRect|LiveValue|Part(?:Bounds|s)|Thumb(?:PositionFrom(?:Bounds|Offset)|Shape)))|UIFontType|Window(?:RegionHit|Shape))|HitTest(?:ScrollBarArrows|Track)|Set(?:Fill|Stroke|TextFill)))|I(?:O(?:BSDNameMatching|C(?:atalogue(?:GetData|ModuleLoaded|Reset|SendData|Terminate)|onnect(?:Add(?:Client|Ref)|Call(?:Async(?:Method|S(?:calarMethod|tructMethod))|Method|S(?:calarMethod|tructMethod))|GetService|MapMemory(?:64)?|Release|Set(?:CFPropert(?:ies|y)|NotificationPort)|Trap(?:0|1|2|3|4|5|6)|UnmapMemory(?:64)?)|reateReceivePort)|DispatchCalloutFromMessage|Iterator(?:IsValid|Next|Reset)|Kit(?:GetBusyState|WaitQuiet)|MasterPort|NotificationPort(?:Create|Destroy|Get(?:MachPort|RunLoopSource)|Set(?:DispatchQueue|ImportanceReceiver))|O(?:bject(?:Co(?:nformsTo|py(?:BundleIdentifierForClass|Class|SuperclassForClass))|Get(?:Class|KernelRetainCount|RetainCount|UserRetainCount)|IsEqualTo|Re(?:lease|tain))|penFirmwarePathMatching)|Registry(?:CreateIterator|Entry(?:Create(?:CFPropert(?:ies|y)|Iterator)|FromPath|Get(?:Child(?:Entry|Iterator)|LocationInPlane|Name(?:InPlane)?|P(?:a(?:rent(?:Entry|Iterator)|th)|roperty)|RegistryEntryID)|I(?:DMatching|nPlane)|Se(?:archCFProperty|tCFPropert(?:ies|y)))|GetRootEntry|IteratorE(?:nterEntry|xitEntry))|S(?:ervice(?:A(?:dd(?:InterestNotification|MatchingNotification|Notification)|uthorize)|Close|Get(?:BusyState|MatchingService(?:s)?)|Match(?:PropertyTable|ing)|NameMatching|O(?:FPathToBSDName|pen(?:AsFileDescriptor)?)|RequestProbe|WaitQuiet)|urface(?:AlignProperty|C(?:opy(?:AllValues|Value)|reate(?:MachPort|XPCObject)?)|DecrementUseCount|Get(?:AllocSize|B(?:aseAddress(?:OfPlane)?|ytesPer(?:Element(?:OfPlane)?|Row(?:OfPlane)?))|Element(?:Height(?:OfPlane)?|Width(?:OfPlane)?)|Height(?:OfPlane)?|ID|P(?:ixelFormat|laneCount|roperty(?:Alignment|Maximum))|Seed|TypeID|UseCount|Width(?:OfPlane)?)|I(?:ncrementUseCount|sInUse)|Lo(?:ck|okup(?:From(?:MachPort|XPCObject))?)|Remove(?:AllValues|Value)|SetValue(?:s)?|Unlock)))|conRef(?:ContainsCGPoint|IntersectsCGRect|To(?:HIShape|IconFamily))|n(?:stallEvent(?:Handler|LoopTimer)|voke(?:AE(?:Coerce(?:DescUPP|PtrUPP)|DisposeExternalUPP|EventHandlerUPP|FilterUPP|IdleUPP)|C(?:a(?:librate(?:EventUPP|UPP)|nCalibrateUPP)|ontrol(?:ActionUPP|EditTextValidationUPP|KeyFilterUPP|UserPane(?:ActivateUPP|DrawUPP|FocusUPP|HitTestUPP|IdleUPP|KeyDownUPP|TrackingUPP)))|D(?:ataBrowser(?:A(?:cceptDragUPP|ddDragItemUPP)|DrawItemUPP|EditItemUPP|GetContextualMenuUPP|HitTestUPP|Item(?:AcceptDragUPP|CompareUPP|D(?:ataUPP|ragRgnUPP)|HelpContentUPP|Notification(?:UPP|WithItemUPP)|ReceiveDragUPP|UPP)|PostProcessDragUPP|ReceiveDragUPP|SelectContextualMenuUPP|TrackingUPP)|ragInputUPP)|E(?:ditUnicodePostUpdateUPP|vent(?:ComparatorUPP|HandlerUPP|Loop(?:IdleTimerUPP|TimerUPP)))|HM(?:ControlContentUPP|Menu(?:ItemContentUPP|TitleContentUPP)|WindowContentUPP)|I(?:con(?:ActionUPP|GetterUPP)|ndexToUCStringUPP)|ModalFilter(?:UPP|YDUPP)|N(?:ColorChangedUPP|MUPP)|OS(?:A(?:ActiveUPP|CreateAppleEventUPP|SendUPP)|L(?:A(?:ccessorUPP|djustMarksUPP)|Co(?:mpareUPP|untUPP)|DisposeTokenUPP|Get(?:ErrDescUPP|MarkTokenUPP)|MarkUPP))|TXN(?:ActionNameMapperUPP|ContextualMenuSetupUPP|FindUPP|ScrollInfoUPP)|U(?:nicodeToTextFallbackUPP|serItemUPP)))|s(?:EventInQueue|IconRefMaskEmpty|SecureEventInputEnabled|UserCancelEventRef))|JS(?:C(?:heckScriptSyntax|lass(?:Create|Re(?:lease|tain))|ontextG(?:etG(?:lobal(?:Context|Object)|roup)|roup(?:Create|Re(?:lease|tain))))|EvaluateScript|G(?:arbageCollect|lobalContext(?:Create(?:InGroup)?|Re(?:lease|tain)))|Object(?:C(?:allAs(?:Constructor|Function)|opyPropertyNames)|DeleteProperty|GetPr(?:ivate|o(?:perty(?:AtIndex)?|totype))|HasProperty|Is(?:Constructor|Function)|Make(?:Array|Constructor|Date|Error|Function(?:WithCallback)?|RegExp)?|SetPr(?:ivate|o(?:perty(?:AtIndex)?|totype)))|PropertyNameA(?:ccumulatorAddName|rray(?:Get(?:Count|NameAtIndex)|Re(?:lease|tain)))|String(?:C(?:opyCFString|reateWith(?:C(?:FString|haracters)|UTF8CString))|Get(?:CharactersPtr|Length|MaximumUTF8CStringSize|UTF8CString)|IsEqual(?:ToUTF8CString)?|Re(?:lease|tain))|Value(?:CreateJSONString|GetType|Is(?:Boolean|Equal|InstanceOfConstructor|Nu(?:ll|mber)|Object(?:OfClass)?|Stri(?:ctEqual|ng)|Undefined)|Make(?:Boolean|FromJSONString|Nu(?:ll|mber)|String|Undefined)|Protect|To(?:Boolean|Number|Object|StringCopy)|Unprotect))|KBGetLayoutType|L(?:MGetK(?:bd(?:Last|Type)|ey(?:RepThresh|Thresh))|S(?:C(?:anURLAcceptURL|opy(?:A(?:llRoleHandlersForContentType|pplicationURLsForURL)|DefaultRoleHandlerForContentType))|Open(?:CFURLRef|FromURLSpec)|RegisterURL|SetDefault(?:HandlerForURLScheme|RoleHandlerForContentType))|o(?:cale(?:Operation(?:CountNames|Get(?:IndName|Name))|Ref(?:FromL(?:angOrRegionCode|ocaleString)|GetPartString)|StringToLangAndRegionCodes)|ngDoubleTo(?:SInt64|UInt64)))|M(?:D(?:CopyLabel(?:Kinds|WithUUID|s(?:MatchingExpression|WithKind))|Item(?:C(?:opy(?:Attribute(?:List|Names|s)?|Labels)|reate(?:WithURL)?)|GetTypeID|RemoveLabel|SetLabel|sCreateWithURLs)|Label(?:C(?:opyAttribute(?:Name)?|reate)|Delete|GetTypeID|SetAttributes)|Query(?:C(?:opy(?:QueryString|SortingAttributes|Value(?:ListAttributes|sOfAttribute))|reate(?:ForItems|Subset)?)|DisableUpdates|E(?:nableUpdates|xecute)|Get(?:AttributeValueOfResultAtIndex|BatchingParameters|CountOfResultsWithAttributeValue|IndexOfResult|Result(?:AtIndex|Count)|SortOptionFlagsForAttribute|TypeID)|IsGatheringComplete|S(?:et(?:BatchingParameters|Create(?:ResultFunction|ValueFunction)|DispatchQueue|MaxCount|S(?:earchScope|ort(?:Comparator(?:Block)?|O(?:ptionFlagsForAttribute|rder))))|top))|SchemaCopy(?:A(?:llAttributes|ttributesForContentType)|Display(?:DescriptionForAttribute|NameForAttribute)|MetaAttributesForAttribute))|IDI(?:Client(?:Create|Dispose)|De(?:stinationCreate|viceGet(?:Entity|NumberOfEntities))|En(?:dpoint(?:Dispose|GetEntity)|tityGet(?:De(?:stination|vice)|NumberOf(?:Destinations|Sources)|Source))|FlushOutput|Get(?:De(?:stination|vice)|ExternalDevice|NumberOf(?:De(?:stinations|vices)|ExternalDevices|Sources)|Source)|InputPortCreate|O(?:bject(?:FindByUniqueID|Get(?:D(?:ataProperty|ictionaryProperty)|IntegerProperty|Properties|StringProperty)|RemoveProperty|Set(?:D(?:ataProperty|ictionaryProperty)|IntegerProperty|StringProperty))|utputPortCreate)|P(?:acket(?:List(?:Add|Init)|Next)|ort(?:ConnectSource|Dis(?:connectSource|pose)))|Re(?:ceived|start)|S(?:end(?:Sysex)?|ourceCreate))|akeVoiceSpec|usic(?:Device(?:MIDIEvent|S(?:t(?:artNote|opNote)|ysEx))|EventIterator(?:DeleteEvent|GetEventInfo|Has(?:CurrentEvent|NextEvent|PreviousEvent)|NextEvent|PreviousEvent|Se(?:ek|tEvent(?:Info|Time)))|Player(?:Get(?:BeatsForHostTime|HostTimeForBeats|PlayRateScalar|Sequence|Time)|IsPlaying|Preroll|S(?:et(?:PlayRateScalar|Sequence|Time)|t(?:art|op)))|Sequence(?:B(?:arBeatTimeToBeats|eatsToBarBeatTime)|DisposeTrack|File(?:Create(?:Data)?|Load(?:Data)?)|Get(?:AUGraph|BeatsForSeconds|In(?:dTrack|foDictionary)|S(?:MPTEResolution|e(?:condsForBeats|quenceType))|T(?:empoTrack|rack(?:Count|Index)))|NewTrack|Reverse|Set(?:AUGraph|MIDIEndpoint|S(?:MPTEResolution|equenceType)|UserCallback))|Track(?:C(?:lear|opyInsert|ut)|Get(?:Dest(?:MIDIEndpoint|Node)|Property|Sequence)|M(?:erge|oveEvents)|New(?:AUPresetEvent|Extended(?:NoteEvent|TempoEvent)|M(?:IDI(?:ChannelEvent|NoteEvent|RawDataEvent)|etaEvent)|ParameterEvent|UserEvent)|Set(?:Dest(?:MIDIEndpoint|Node)|Property))))|N(?:PickColor|X(?:Convert(?:Host(?:DoubleToSwapped|FloatToSwapped)|Swapped(?:DoubleToHost|FloatToHost))|HostByteOrder|Swap(?:Big(?:DoubleToHost|FloatToHost|IntToHost|Long(?:LongToHost|ToHost)|ShortToHost)|Double|Float|Host(?:DoubleTo(?:Big|Little)|FloatTo(?:Big|Little)|IntTo(?:Big|Little)|Long(?:LongTo(?:Big|Little)|To(?:Big|Little))|ShortTo(?:Big|Little))|Int|L(?:ittle(?:DoubleToHost|FloatToHost|IntToHost|Long(?:LongToHost|ToHost)|ShortToHost)|ong(?:Long)?)|Short))|e(?:arestMacTextEncodings|w(?:AE(?:Coerce(?:DescUPP|PtrUPP)|DisposeExternalUPP|EventHandlerUPP|FilterUPP|IdleUPP)|C(?:a(?:librate(?:EventUPP|UPP)|nCalibrateUPP)|ontrol(?:ActionUPP|EditTextValidationUPP|KeyFilterUPP|UserPane(?:ActivateUPP|DrawUPP|FocusUPP|HitTestUPP|IdleUPP|KeyDownUPP|TrackingUPP)))|D(?:ataBrowser(?:A(?:cceptDragUPP|ddDragItemUPP)|DrawItemUPP|EditItemUPP|GetContextualMenuUPP|HitTestUPP|Item(?:AcceptDragUPP|CompareUPP|D(?:ataUPP|ragRgnUPP)|HelpContentUPP|Notification(?:UPP|WithItemUPP)|ReceiveDragUPP|UPP)|PostProcessDragUPP|ReceiveDragUPP|SelectContextualMenuUPP|TrackingUPP)|ragInputUPP)|E(?:ditUnicodePostUpdateUPP|vent(?:ComparatorUPP|HandlerUPP|Loop(?:IdleTimerUPP|TimerUPP)))|HM(?:ControlContentUPP|Menu(?:ItemContentUPP|TitleContentUPP)|WindowContentUPP)|I(?:con(?:ActionUPP|GetterUPP)|ndexToUCStringUPP)|M(?:odalFilter(?:UPP|YDUPP)|usic(?:EventIterator|Player|Sequence))|N(?:ColorChangedUPP|MUPP)|OS(?:A(?:ActiveUPP|CreateAppleEventUPP|SendUPP)|L(?:A(?:ccessorUPP|djustMarksUPP)|Co(?:mpareUPP|untUPP)|DisposeTokenUPP|Get(?:ErrDescUPP|MarkTokenUPP)|MarkUPP))|SpeechChannel|TXN(?:ActionNameMapperUPP|ContextualMenuSetupUPP|FindUPP|ScrollInfoUPP)|U(?:nicodeToTextFallbackUPP|serItemUPP))|xtAudioFileRegion)|um(?:AudioFileMarkersToNumBytes|BytesToNumAudioFileMarkers))|OS(?:A(?:A(?:ddStorageType|vailableDialect(?:CodeList|s))|Co(?:erce(?:FromDesc|ToDesc)|mpile(?:Execute)?|py(?:DisplayString|ID|S(?:cript(?:ingDefinition(?:FromURL)?)?|ourceString)))|D(?:isp(?:lay|ose)|o(?:Event|Script(?:File)?))|Execute(?:Event)?|Ge(?:nericToRealID|t(?:ActiveProc|C(?:reateProc|urrentDialect)|D(?:efaultScriptingComponent|ialectInfo)|Handler(?:Names)?|Property(?:Names)?|ResumeDispatchProc|S(?:cript(?:DataFromURL|Info|ingComponent(?:FromStored)?)|endProc|ource|torageType|ysTerminology)))|Load(?:Execute(?:File)?|File|ScriptData)?|MakeContext|Re(?:alToGenericID|moveStorageType)|S(?:cript(?:Error|ingComponentName)|et(?:ActiveProc|C(?:reateProc|urrentDialect)|Default(?:ScriptingComponent|Target)|Handler|Property|ResumeDispatchProc|S(?:criptInfo|endProc))|t(?:artRecording|o(?:pRecording|re(?:File)?)))|tomic(?:Dequeue|Enqueue|Fifo(?:Dequeue|Enqueue)))|GetNotificationFromMessage)|P(?:M(?:C(?:GImageCreateWithEPSDataProvider|opy(?:AvailablePPDs|LocalizedPPD|P(?:PDData|ageFormat|rintSettings))|reate(?:GenericPrinter|P(?:ageFormat(?:WithPMPaper)?|rintSettings)|Session))|Get(?:AdjustedPa(?:geRect|perRect)|Co(?:llate|pies)|Duplex|FirstPage|LastPage|Orientation|Page(?:Format(?:ExtendedData|Paper)|Range)|Scale|UnadjustedPa(?:geRect|perRect))|P(?:a(?:geFormat(?:Create(?:DataRepresentation|WithDataRepresentation)|GetPrinterID)|per(?:Create(?:Custom|LocalizedName)|Get(?:Height|ID|Margins|P(?:PDPaperName|rinterID)|Width)|IsCustom))|r(?:eset(?:C(?:opyName|reatePrintSettings)|GetAttributes)|int(?:Settings(?:C(?:opy(?:AsDictionary|Keys)|reate(?:DataRepresentation|WithDataRepresentation))|Get(?:JobName|Value)|Set(?:JobName|Value)|ToOptions(?:WithPrinterAndPageFormat)?)|er(?:C(?:opy(?:De(?:scriptionURL|viceURI)|HostName|Presets|State)|reateFromPrinterID)|Get(?:CommInfo|Driver(?:Creator|ReleaseInfo)|I(?:D|ndexedPrinterResolution)|L(?:anguageInfo|ocation)|M(?:akeAndModelName|imeTypes)|Name|OutputResolution|P(?:aperList|rinterResolutionCount)|State)|Is(?:Default|Favorite|PostScript(?:Capable|Printer)|Remote)|PrintWith(?:File|Provider)|Se(?:ndCommand|t(?:Default|OutputResolution))|WritePostScriptToURL))))|Re(?:lease|tain)|Se(?:rver(?:CreatePrinterList|LaunchPrinterBrowser)|ssion(?:Begin(?:CGDocumentNoDialog|PageNoDialog)|C(?:opy(?:Destination(?:Format|Location)|OutputFormatList)|reateP(?:ageFormatList|rinterList))|DefaultP(?:ageFormat|rintSettings)|E(?:nd(?:DocumentNoDialog|PageNoDialog)|rror)|Get(?:C(?:GGraphicsContext|urrentPrinter)|D(?:ataFromSession|estinationType))|Set(?:CurrentPMPrinter|D(?:ataInSession|estination)|Error)|ValidateP(?:ageFormat|rintSettings))|t(?:Co(?:llate|pies)|Duplex|FirstPage|LastPage|Orientation|Page(?:FormatExtendedData|Range)|Scale))|Workflow(?:CopyItems|SubmitPDFWith(?:Options|Settings)))|a(?:steboard(?:C(?:lear|opy(?:ItemFlavor(?:Data|s)|Name|PasteLocation)|reate)|Get(?:Item(?:Count|FlavorFlags|Identifier)|TypeID)|PutItemFlavor|ResolvePromises|S(?:etP(?:asteLocation|romiseKeeper)|ynchronize))|useSpeechAt)|lotIconRefInContext|o(?:pSymbolicHotKeyMode|stEventToQueue)|rocessHICommand|ushSymbolicHotKeyMode)|Q(?:LThumbnailImageCreate|u(?:eryUnicodeMappings|itEventLoop))|R(?:e(?:ceiveNextEvent|gisterEventHotKey|leaseEvent|moveEvent(?:FromQueue|Handler|LoopTimer|Parameter|TypesFromHandler)|s(?:et(?:TextToUnicodeInfo|UnicodeToText(?:Info|RunInfo))|olveDefaultTextEncoding)|tainEvent|vertTextEncodingToScriptInfo)|unCurrentEventLoop)|S(?:32Set|64(?:A(?:dd|nd)|Bitwise(?:And|Eor|Not|Or)|Div(?:ide)?|Eor|M(?:ax|in|od|ultiply)|N(?:egate|ot)|Or|S(?:et(?:U)?|hift(?:Left|Right)|ubtract))|C(?:Bond(?:Interface(?:C(?:opy(?:A(?:ll|vailableMemberInterfaces)|Status)|reate)|Get(?:MemberInterfaces|Options)|Remove|Set(?:LocalizedDisplayName|MemberInterfaces|Options))|StatusGet(?:InterfaceStatus|MemberInterfaces|TypeID))|CopyLastError|DynamicStore(?:Add(?:TemporaryValue|Value)|C(?:opy(?:Co(?:mputerName|nsoleUser)|KeyList|Loca(?:lHostName|tion)|Multiple|NotifiedKeys|Proxies|Value)|reate(?:RunLoopSource|WithOptions)?)|GetTypeID|KeyCreate(?:Co(?:mputerName|nsoleUser)|HostNames|Location|Network(?:GlobalEntity|Interface(?:Entity)?|ServiceEntity)|Proxies)?|NotifyValue|RemoveValue|Set(?:DispatchQueue|Multiple|NotificationKeys|Value))|Error(?:String)?|Network(?:Connection(?:C(?:opy(?:ExtendedStatus|S(?:erviceID|tatistics)|User(?:Options|Preferences))|reateWithServiceID)|Get(?:Status|TypeID)|S(?:cheduleWithRunLoop|etDispatchQueue|t(?:art|op))|UnscheduleFromRunLoop)|Interface(?:C(?:opy(?:All|M(?:TU|edia(?:Options|SubType(?:Options|s))))|reateWithInterface)|ForceConfigurationRefresh|Get(?:BSDName|Configuration|ExtendedConfiguration|HardwareAddressString|Interface(?:Type)?|LocalizedDisplayName|Supported(?:InterfaceTypes|ProtocolTypes)|TypeID)|Set(?:Configuration|ExtendedConfiguration|M(?:TU|ediaOptions)))|Protocol(?:Get(?:Configuration|Enabled|ProtocolType|TypeID)|Set(?:Configuration|Enabled))|Reachability(?:CreateWith(?:Address(?:Pair)?|Name)|Get(?:Flags|TypeID)|S(?:cheduleWithRunLoop|et(?:Callback|DispatchQueue))|UnscheduleFromRunLoop)|Se(?:rvice(?:AddProtocolType|C(?:opy(?:All|Protocol(?:s)?)?|reate)|EstablishDefaultConfiguration|Get(?:Enabled|Interface|Name|ServiceID|TypeID)|Remove(?:ProtocolType)?|Set(?:Enabled|Name))|t(?:AddService|C(?:o(?:ntainsInterface|py(?:All|Current|Services)?)|reate)|Get(?:Name|Se(?:rviceOrder|tID)|TypeID)|Remove(?:Service)?|Set(?:Current|Name|ServiceOrder))))|Preferences(?:A(?:ddValue|pplyChanges)|C(?:o(?:mmitChanges|pyKeyList)|reate(?:WithAuthorization)?)|Get(?:Signature|TypeID|Value)|Lock|Path(?:CreateUniqueChild|Get(?:Link|Value)|RemoveValue|Set(?:Link|Value))|RemoveValue|S(?:cheduleWithRunLoop|et(?:C(?:allback|omputerName)|DispatchQueue|LocalHostName|Value)|ynchronize)|Un(?:lock|scheduleFromRunLoop))|VLANInterface(?:C(?:opyA(?:ll|vailablePhysicalInterfaces)|reate)|Get(?:Options|PhysicalInterface|Tag)|Remove|Set(?:LocalizedDisplayName|Options|PhysicalInterfaceAndTag)))|Int64To(?:LongDouble|UInt64|Wide)|K(?:Document(?:C(?:opyURL|reate(?:WithURL)?)|Get(?:Name|Parent|SchemeName|TypeID))|Index(?:AddDocument(?:WithText)?|C(?:lose|o(?:mpact|py(?:Document(?:ForDocumentID|IDArrayForTermID|Properties|RefsForDocumentIDs|URLsForDocumentIDs)|InfoForDocumentIDs|Term(?:IDArrayForDocumentID|StringForTermID)))|reateWith(?:MutableData|URL))|DocumentIterator(?:C(?:opyNext|reate)|GetTypeID)|Flush|Get(?:AnalysisProperties|Document(?:Count|ID|State|Term(?:Count|Frequency))|IndexType|Maximum(?:BytesBeforeFlush|DocumentID|TermID)|T(?:erm(?:DocumentCount|IDForTermString)|ypeID))|MoveDocument|OpenWith(?:Data|MutableData|URL)|Re(?:moveDocument|nameDocument)|Set(?:DocumentProperties|MaximumBytesBeforeFlush))|LoadDefaultExtractorPlugIns|S(?:earch(?:C(?:ancel|reate)|FindMatches|GetTypeID)|ummary(?:C(?:opy(?:Paragraph(?:AtIndex|SummaryString)|Sentence(?:AtIndex|SummaryString))|reateWithString)|Get(?:Paragraph(?:Count|SummaryInfo)|Sentence(?:Count|SummaryInfo)|TypeID))))|e(?:c(?:A(?:CL(?:C(?:opy(?:Authorizations|Contents)|reateWithSimpleContents)|GetTypeID|Remove|SetContents|UpdateAuthorizations)|ccess(?:C(?:opy(?:ACLList|MatchingACLList|OwnerAndACL)|reate(?:WithOwnerAndACL)?)|GetTypeID)|ddSharedWebCredential)|C(?:ertificate(?:AddToKeychain|C(?:opy(?:CommonName|Data|EmailAddresses|LongDescription|Preferred|S(?:hortDescription|ubjectSummary)|Values)|reateWithData)|GetTypeID|SetPreferred)|o(?:de(?:C(?:heckValidity(?:WithErrors)?|opy(?:DesignatedRequirement|GuestWithAttributes|Host|Path|S(?:elf|igningInformation|taticCode)))|GetTypeID|MapMemory)|pyErrorMessageString)|reateSharedWebCredentialPassword)|D(?:ec(?:odeTransformCreate|ryptTransform(?:Create|GetTypeID))|igestTransform(?:Create|GetTypeID))|Enc(?:odeTransformCreate|ryptTransform(?:Create|GetTypeID))|GroupTransformGetTypeID|I(?:dentity(?:C(?:opy(?:Certificate|Pr(?:eferred|ivateKey)|SystemIdentity)|reateWithCertificate)|GetTypeID|Set(?:Preferred|SystemIdentity))|tem(?:Add|CopyMatching|Delete|Export|Import|Update))|Key(?:CreateFromData|DeriveFromPassword|Ge(?:nerate(?:Pair(?:Async)?|Symmetric)|t(?:BlockSize|TypeID))|UnwrapSymmetric|WrapSymmetric|chain(?:A(?:dd(?:Callback|GenericPassword|InternetPassword)|ttributeInfoForItemID)|C(?:opy(?:D(?:efault|omain(?:Default|SearchList))|Se(?:archList|ttings))|reate)|Delete|F(?:ind(?:GenericPassword|InternetPassword)|reeAttributeInfo)|Get(?:P(?:ath|referenceDomain)|Status|TypeID|UserInteractionAllowed|Version)|Item(?:C(?:opy(?:A(?:ccess|ttributesAndData)|Content|FromPersistentReference|Keychain)|reate(?:Copy|FromContent|PersistentReference))|Delete|Free(?:AttributesAndData|Content)|GetTypeID|Modify(?:AttributesAndData|Content)|SetAccess)|Lock(?:All)?|Open|RemoveCallback|Set(?:D(?:efault|omain(?:Default|SearchList))|PreferenceDomain|Se(?:archList|ttings)|UserInteractionAllowed)|Unlock))|P(?:KCS12Import|olicy(?:C(?:opyProperties|reate(?:BasicX509|SSL))|GetTypeID))|R(?:andomCopyBytes|equ(?:estSharedWebCredential|irement(?:C(?:opy(?:Data|String)|reateWith(?:Data|String(?:AndErrors)?))|GetTypeID)))|S(?:ignTransformCreate|taticCode(?:C(?:heckValidity(?:WithErrors)?|reateWithPath(?:AndAttributes)?)|GetTypeID))|T(?:ask(?:C(?:opy(?:SigningIdentifier|Value(?:ForEntitlement|sForEntitlements))|reate(?:FromSelf|WithAuditToken))|Get(?:CodeSignStatus|TypeID))|r(?:ansform(?:C(?:o(?:nnectTransforms|pyExternalRepresentation)|reate(?:FromExternalRepresentation|GroupTransform|ReadTransformWithReadStream)?|ustom(?:GetAttribute|SetAttribute))|Execute(?:Async)?|FindByName|Get(?:Attribute|TypeID)|NoData|PushbackAttribute|Register|Set(?:Attribute(?:Action)?|DataAction|TransformAction))|ust(?:C(?:opy(?:AnchorCertificates|CustomAnchorCertificates|P(?:olicies|roperties|ublicKey))|reateWithCertificates)|Get(?:Certificate(?:AtIndex|Count)|T(?:rustResult|ypeID)|VerifyTime)|Set(?:AnchorCertificates(?:Only)?|Options|Policies|VerifyDate|tings(?:C(?:opy(?:Certificates|ModificationDate|TrustSettings)|reateExternalRepresentation)|ImportExternalRepresentation|RemoveTrustSettings|SetTrustSettings))|edApplicationGetTypeID)))|VerifyTransformCreate)|ndEventToEventTarget(?:WithOptions)?|ssion(?:Create|GetInfo)|t(?:AudioUnitParameterDisplayType|Event(?:LoopTimerNextFireTime|Parameter|Time)|F(?:allbackUnicodeToText(?:Run)?|ontInfoForSelection)|IconFamilyData|S(?:peech(?:P(?:itch|roperty)|Rate)|ystemUIMode)))|pe(?:akCFString|ech(?:Busy(?:SystemWide)?|ManagerVersion|Synthesis(?:RegisterModuleURL|UnregisterModuleURL)))|topSpeech(?:At)?)|T(?:EC(?:C(?:lear(?:ConverterContextInfo|SnifferContextInfo)|o(?:nvertText(?:ToMultipleEncodings)?|pyTextEncodingInternetNameAndMIB|unt(?:Available(?:Sniffers|TextEncodings)|D(?:estinationTextEncodings|irectTextEncodingConversions)|MailTextEncodings|SubTextEncodings|WebTextEncodings))|reate(?:Converter(?:FromPath)?|OneToManyConverter|Sniffer))|Dispose(?:Converter|Sniffer)|Flush(?:MultipleEncodings|Text)|Get(?:Available(?:Sniffers|TextEncodings)|D(?:estinationTextEncodings|irectTextEncodingConversions)|EncodingList|Info|MailTextEncodings|SubTextEncodings|TextEncoding(?:FromInternetName(?:OrMIB)?|InternetName)|WebTextEncodings)|S(?:etBasicOptions|niffTextEncoding))|IS(?:C(?:opy(?:Current(?:ASCIICapableKeyboard(?:InputSource|LayoutInputSource)|Keyboard(?:InputSource|LayoutInputSource))|Input(?:MethodKeyboardLayoutOverride|SourceForLanguage))|reate(?:ASCIICapableInputSourceList|InputSourceList))|D(?:eselectInputSource|isableInputSource)|EnableInputSource|GetInputSourceProperty|InputSourceGetTypeID|RegisterInputSource|Se(?:lectInputSource|tInputMethodKeyboardLayoutOverride))|SM(?:Get(?:ActiveDocument|DocumentProperty)|RemoveDocumentProperty|SetDocumentProperty)|r(?:ans(?:formProcessType|lation(?:C(?:opy(?:DestinationType|SourceType)|reate(?:WithSourceArray)?)|GetT(?:ranslationFlags|ypeID)|PerformFor(?:Data|File|URL)))|uncateFor(?:TextToUnicode|UnicodeToText)))|U(?:32SetU|64(?:A(?:dd|nd)|Bitwise(?:And|Eor|Not|Or)|Div(?:ide)?|Eor|M(?:ax|od|ultiply)|Not|Or|S(?:et(?:U)?|hift(?:Left|Right)|ubtract))|AZoom(?:ChangeFocus|Enabled)|C(?:C(?:o(?:mpare(?:CollationKeys|Text(?:Default|NoLocale)?)|nvert(?:CFAbsoluteTimeTo(?:LongDateTime|Seconds|UTCDateTime)|LongDateTimeToCFAbsoluteTime|SecondsToCFAbsoluteTime|UTCDateTimeToCFAbsoluteTime))|reateCollator)|DisposeCollator|Get(?:C(?:harProperty|ollationKey)|UnicodeScalarValueForSurrogatePair)|IsSurrogate(?:HighCharacter|LowCharacter)|KeyTranslate|TypeSelect(?:AddKeyToSelector|C(?:ompare|reateSelector)|F(?:indItem|lushSelectorData)|ReleaseSelector|W(?:alkList|ouldResetBuffer)))|Int64To(?:LongDouble|SInt64|UnsignedWide)|T(?:CreateStringForOSType|GetOSTypeFromString|Type(?:C(?:o(?:nformsTo|py(?:De(?:clar(?:ation|ingBundleURL)|scription)|PreferredTagWithClass))|reate(?:AllIdentifiersForTag|PreferredIdentifierForTag))|Equal))|n(?:registerEventHotKey|signedWideToUInt64)|pgradeScriptInfoToTextEncoding|seSpeechDictionary)|WideToSInt64|a(?:c(?:cept|l_(?:add_(?:flag_np|perm)|c(?:alc_mask|lear_(?:flags_np|perms)|opy_(?:e(?:ntry|xt(?:_native)?)|int(?:_native)?)|reate_entry(?:_np)?)|d(?:elete_(?:def_file|entry|flag_np|perm)|up)|fr(?:ee|om_text)|get_(?:entry|f(?:d(?:_np)?|ile|lag(?:_np|set_np))|link_np|perm(?:_np|set(?:_mask_np)?)|qualifier|tag_type)|init|maximal_permset_mask_np|s(?:et_(?:f(?:d(?:_np)?|ile|lagset_np)|link_np|permset(?:_mask_np)?|qualifier|tag_type)|ize)|to_text|valid(?:_(?:f(?:d_np|ile_np)|link_np))?)|t_(?:get_state|set_state))|udit(?:_session_(?:join|port|self)|ctl|on)?)|bind|c(?:a(?:bs(?:f|l)?|cos(?:f|h(?:f|l)?|l)?|lloc|rg(?:f|l)?|sin(?:f|h(?:f|l)?|l)?|tan(?:f|h(?:f|l)?|l)?)|cos(?:f|h(?:f|l)?|l)?|exp(?:f|l)?|imag(?:f|l)?|lo(?:ck_(?:get_res|s(?:et_(?:attributes|res|time)|leep(?:_trap)?))|g(?:f|l)?)|on(?:j(?:f|l)?|nect)|p(?:ow(?:f|l)?|roj(?:f|l)?)|real(?:f|l)?|s(?:in(?:f|h(?:f|l)?|l)?|qrt(?:f|l)?|sm(?:AlgToOid|OidToAlg|Perror))|t(?:an(?:f|h(?:f|l)?|l)?|ermid))|d(?:e(?:bug_control_port_for_pid|c2numl)|igittoint)|etap_trace_thread|f(?:e(?:clearexcept|get(?:e(?:nv|xceptflag)|round)|holdexcept|raiseexcept|set(?:e(?:nv|xceptflag)|round)|testexcept|updateenv)|ree)|g(?:et(?:au(?:dit_addr|id)|peername|sock(?:name|opt))|l(?:A(?:c(?:cum|tive(?:StencilFaceEXT|Texture(?:ARB)?))|lphaFunc|r(?:eTexturesResident|rayElement)|ttach(?:ObjectARB|Shader))|B(?:egin(?:ConditionalRenderNV|Query(?:ARB)?|TransformFeedbackEXT)?|i(?:nd(?:AttribLocation(?:ARB)?|Buffer(?:ARB|BaseEXT|OffsetEXT|RangeEXT)?|Fra(?:gDataLocationEXT|mebuffer(?:EXT)?)|ProgramARB|Renderbuffer(?:EXT)?|Texture|VertexArrayAPPLE)|tmap)|l(?:end(?:Color(?:EXT)?|Equation(?:EXT|Separate(?:ATI|EXT)?)?|Func(?:Separate(?:EXT)?)?)|itFramebuffer(?:EXT)?)|uffer(?:Data(?:ARB)?|ParameteriAPPLE|SubData(?:ARB)?))|C(?:allList(?:s)?|heckFramebufferStatus(?:EXT)?|l(?:ampColorARB|ear(?:Accum|Color(?:I(?:iEXT|uiEXT))?|Depth|Index|Stencil)?|i(?:ent(?:ActiveTexture(?:ARB)?|WaitSync)|pPlane))|o(?:lor(?:3(?:b(?:v)?|d(?:v)?|f(?:v)?|i(?:v)?|s(?:v)?|u(?:b(?:v)?|i(?:v)?|s(?:v)?))|4(?:b(?:v)?|d(?:v)?|f(?:v)?|i(?:v)?|s(?:v)?|u(?:b(?:v)?|i(?:v)?|s(?:v)?))|Ma(?:sk(?:IndexedEXT)?|terial)|Pointer|SubTable|Table(?:Parameter(?:fv|iv))?)|mp(?:ileShader(?:ARB)?|ressedTex(?:Image(?:1D(?:ARB)?|2D(?:ARB)?|3D(?:ARB)?)|SubImage(?:1D(?:ARB)?|2D(?:ARB)?|3D(?:ARB)?)))|nvolution(?:Filter(?:1D|2D)|Parameter(?:f(?:v)?|i(?:v)?))|py(?:Co(?:lor(?:SubTable|Table)|nvolutionFilter(?:1D|2D))|Pixels|Tex(?:Image(?:1D|2D)|SubImage(?:1D|2D|3D))))|reate(?:Program(?:ObjectARB)?|Shader(?:ObjectARB)?)|ullFace)|D(?:e(?:lete(?:Buffers(?:ARB)?|F(?:encesAPPLE|ramebuffers(?:EXT)?)|Lists|ObjectARB|Program(?:sARB)?|Queries(?:ARB)?|Renderbuffers(?:EXT)?|S(?:hader|ync)|Textures|VertexArraysAPPLE)|pth(?:BoundsEXT|Func|Mask|Range)|tach(?:ObjectARB|Shader))|isable(?:ClientState|IndexedEXT|VertexAttribA(?:PPLE|rray(?:ARB)?))?|raw(?:Arrays(?:InstancedARB)?|Buffer(?:s(?:ARB)?)?|Element(?:ArrayAPPLE|s(?:BaseVertex|Instanced(?:ARB|BaseVertex))?)|Pixels|RangeElement(?:ArrayAPPLE|s(?:BaseVertex|EXT)?)))|E(?:dgeFlag(?:Pointer|v)?|lementPointerAPPLE|n(?:able(?:ClientState|IndexedEXT|VertexAttribA(?:PPLE|rray(?:ARB)?))?|d(?:ConditionalRenderNV|List|Query(?:ARB)?|TransformFeedbackEXT)?)|val(?:Coord(?:1(?:d(?:v)?|f(?:v)?)|2(?:d(?:v)?|f(?:v)?))|Mesh(?:1|2)|Point(?:1|2)))|F(?:e(?:edbackBuffer|nceSync)|inish(?:FenceAPPLE|ObjectAPPLE|RenderAPPLE)?|lush(?:MappedBufferRangeAPPLE|RenderAPPLE|VertexArrayRangeAPPLE)?|og(?:Coord(?:Pointer(?:EXT)?|d(?:EXT|v(?:EXT)?)?|f(?:EXT|v(?:EXT)?)?)|f(?:v)?|i(?:v)?)|r(?:amebuffer(?:Renderbuffer(?:EXT)?|Texture(?:1D(?:EXT)?|2D(?:EXT)?|3D(?:EXT)?|EXT|FaceEXT|Layer(?:EXT)?))|ontFace|ustum))|Ge(?:n(?:Buffers(?:ARB)?|F(?:encesAPPLE|ramebuffers(?:EXT)?)|Lists|ProgramsARB|Queries(?:ARB)?|Renderbuffers(?:EXT)?|Textures|VertexArraysAPPLE|erateMipmap(?:EXT)?)|t(?:A(?:ctive(?:Attrib(?:ARB)?|Uniform(?:ARB)?)|tt(?:ached(?:ObjectsARB|Shaders)|ribLocation(?:ARB)?))|B(?:oolean(?:IndexedvEXT|v)|uffer(?:P(?:arameteriv(?:ARB)?|ointerv(?:ARB)?)|SubData(?:ARB)?))|C(?:lipPlane|o(?:lorTable(?:Parameter(?:fv|iv))?|mpressedTexImage(?:ARB)?|nvolution(?:Filter|Parameter(?:fv|iv))))|Doublev|Error|F(?:loatv|ra(?:gDataLocationEXT|mebufferAttachmentParameteriv(?:EXT)?))|H(?:andleARB|istogram(?:Parameter(?:fv|iv))?)|In(?:foLogARB|teger(?:64v|IndexedvEXT|v))|Light(?:fv|iv)|M(?:a(?:p(?:dv|fv|iv)|terial(?:fv|iv))|inmax(?:Parameter(?:fv|iv))?)|Object(?:LabelEXT|Parameter(?:fvARB|ivA(?:PPLE|RB)))|P(?:ixelMap(?:fv|u(?:iv|sv))|o(?:interv|lygonStipple)|rogram(?:EnvParameter(?:dvARB|fvARB)|InfoLog|LocalParameter(?:dvARB|fvARB)|StringARB|iv(?:ARB)?))|Query(?:Object(?:i(?:64vEXT|v(?:ARB)?)|ui(?:64vEXT|v(?:ARB)?))|iv(?:ARB)?)|RenderbufferParameteriv(?:EXT)?|S(?:eparableFilter|hader(?:InfoLog|Source(?:ARB)?|iv)|tring|ynciv)|T(?:ex(?:Env(?:fv|iv)|Gen(?:dv|fv|iv)|Image|LevelParameter(?:fv|iv)|Parameter(?:I(?:ivEXT|uivEXT)|PointervAPPLE|fv|iv))|ransformFeedbackVaryingEXT)|Uniform(?:BufferSizeEXT|Location(?:ARB)?|OffsetEXT|fv(?:ARB)?|iv(?:ARB)?|uivEXT)|VertexAttrib(?:I(?:ivEXT|uivEXT)|Pointerv(?:ARB)?|dv(?:ARB)?|fv(?:ARB)?|iv(?:ARB)?)))|Hi(?:nt|stogram)|I(?:n(?:dex(?:Mask|Pointer|d(?:v)?|f(?:v)?|i(?:v)?|s(?:v)?|ub(?:v)?)|itNames|sertEventMarkerEXT|terleavedArrays)|s(?:Buffer(?:ARB)?|Enabled(?:IndexedEXT)?|F(?:enceAPPLE|ramebuffer(?:EXT)?)|List|Program(?:ARB)?|Query(?:ARB)?|Renderbuffer(?:EXT)?|S(?:hader|ync)|Texture|VertexA(?:rrayAPPLE|ttribEnabledAPPLE)))|L(?:abelObjectEXT|i(?:ght(?:Model(?:f(?:v)?|i(?:v)?)|f(?:v)?|i(?:v)?)|n(?:e(?:Stipple|Width)|kProgram(?:ARB)?)|stBase)|o(?:ad(?:Identity|Matrix(?:d|f)|Name|TransposeMatrix(?:d(?:ARB)?|f(?:ARB)?))|gicOp))|M(?:a(?:p(?:1(?:d|f)|2(?:d|f)|Buffer(?:ARB)?|Grid(?:1(?:d|f)|2(?:d|f))|VertexAttrib(?:1(?:dAPPLE|fAPPLE)|2(?:dAPPLE|fAPPLE)))|t(?:erial(?:f(?:v)?|i(?:v)?)|rixMode))|inmax|ult(?:Matrix(?:d|f)|TransposeMatrix(?:d(?:ARB)?|f(?:ARB)?)|i(?:Draw(?:Arrays(?:EXT)?|Element(?:ArrayAPPLE|s(?:BaseVertex|EXT)?)|RangeElementArrayAPPLE)|TexCoord(?:1(?:d(?:ARB|v(?:ARB)?)?|f(?:ARB|v(?:ARB)?)?|i(?:ARB|v(?:ARB)?)?|s(?:ARB|v(?:ARB)?)?)|2(?:d(?:ARB|v(?:ARB)?)?|f(?:ARB|v(?:ARB)?)?|i(?:ARB|v(?:ARB)?)?|s(?:ARB|v(?:ARB)?)?)|3(?:d(?:ARB|v(?:ARB)?)?|f(?:ARB|v(?:ARB)?)?|i(?:ARB|v(?:ARB)?)?|s(?:ARB|v(?:ARB)?)?)|4(?:d(?:ARB|v(?:ARB)?)?|f(?:ARB|v(?:ARB)?)?|i(?:ARB|v(?:ARB)?)?|s(?:ARB|v(?:ARB)?)?)))))|N(?:ewList|ormal(?:3(?:b(?:v)?|d(?:v)?|f(?:v)?|i(?:v)?|s(?:v)?)|Pointer))|O(?:bject(?:PurgeableAPPLE|UnpurgeableAPPLE)|rtho)|P(?:assThrough|ixel(?:Map(?:fv|u(?:iv|sv))|Store(?:f|i)|Transfer(?:f|i)|Zoom)|o(?:int(?:Parameter(?:f(?:ARB|v(?:ARB)?)?|i(?:NV|v(?:NV)?)?)|Size(?:PointerAPPLE)?)|lygon(?:Mode|Offset|Stipple)|p(?:Attrib|ClientAttrib|GroupMarkerEXT|Matrix|Name))|r(?:ioritizeTextures|o(?:gram(?:EnvParameter(?:4(?:d(?:ARB|vARB)|f(?:ARB|vARB))|s4fvEXT)|LocalParameter(?:4(?:d(?:ARB|vARB)|f(?:ARB|vARB))|s4fvEXT)|ParameteriEXT|StringARB)|vokingVertex(?:EXT)?))|ush(?:Attrib|ClientAttrib|GroupMarkerEXT|Matrix|Name))|R(?:asterPos(?:2(?:d(?:v)?|f(?:v)?|i(?:v)?|s(?:v)?)|3(?:d(?:v)?|f(?:v)?|i(?:v)?|s(?:v)?)|4(?:d(?:v)?|f(?:v)?|i(?:v)?|s(?:v)?))|e(?:ad(?:Buffer|Pixels)|ct(?:d(?:v)?|f(?:v)?|i(?:v)?|s(?:v)?)|nder(?:Mode|bufferStorage(?:EXT|Multisample(?:EXT)?)?)|set(?:Histogram|Minmax))|otate(?:d|f))|S(?:ampleCoverage(?:ARB)?|c(?:ale(?:d|f)|issor)|e(?:condaryColor(?:3(?:b(?:EXT|v(?:EXT)?)?|d(?:EXT|v(?:EXT)?)?|f(?:EXT|v(?:EXT)?)?|i(?:EXT|v(?:EXT)?)?|s(?:EXT|v(?:EXT)?)?|u(?:b(?:EXT|v(?:EXT)?)?|i(?:EXT|v(?:EXT)?)?|s(?:EXT|v(?:EXT)?)?))|Pointer(?:EXT)?)|lectBuffer|parableFilter2D|tFenceAPPLE)|hade(?:Model|rSource(?:ARB)?)|tencil(?:Func(?:Separate(?:ATI)?)?|Mask(?:Separate)?|Op(?:Separate(?:ATI)?)?)|wapAPPLE)|T(?:e(?:st(?:FenceAPPLE|ObjectAPPLE)|x(?:Coord(?:1(?:d(?:v)?|f(?:v)?|i(?:v)?|s(?:v)?)|2(?:d(?:v)?|f(?:v)?|i(?:v)?|s(?:v)?)|3(?:d(?:v)?|f(?:v)?|i(?:v)?|s(?:v)?)|4(?:d(?:v)?|f(?:v)?|i(?:v)?|s(?:v)?)|Pointer)|Env(?:f(?:v)?|i(?:v)?)|Gen(?:d(?:v)?|f(?:v)?|i(?:v)?)|Image(?:1D|2D|3D)|Parameter(?:I(?:ivEXT|uivEXT)|f(?:v)?|i(?:v)?)|SubImage(?:1D|2D|3D)|ture(?:BarrierNV|RangeAPPLE)))|rans(?:formFeedbackVaryingsEXT|late(?:d|f)))|U(?:n(?:iform(?:1(?:f(?:ARB|v(?:ARB)?)?|i(?:ARB|v(?:ARB)?)?|ui(?:EXT|vEXT))|2(?:f(?:ARB|v(?:ARB)?)?|i(?:ARB|v(?:ARB)?)?|ui(?:EXT|vEXT))|3(?:f(?:ARB|v(?:ARB)?)?|i(?:ARB|v(?:ARB)?)?|ui(?:EXT|vEXT))|4(?:f(?:ARB|v(?:ARB)?)?|i(?:ARB|v(?:ARB)?)?|ui(?:EXT|vEXT))|BufferEXT|Matrix(?:2(?:fv(?:ARB)?|x(?:3fv|4fv))|3(?:fv(?:ARB)?|x(?:2fv|4fv))|4(?:fv(?:ARB)?|x(?:2fv|3fv))))|mapBuffer(?:ARB)?)|seProgram(?:ObjectARB)?)|V(?:alidateProgram(?:ARB)?|ertex(?:2(?:d(?:v)?|f(?:v)?|i(?:v)?|s(?:v)?)|3(?:d(?:v)?|f(?:v)?|i(?:v)?|s(?:v)?)|4(?:d(?:v)?|f(?:v)?|i(?:v)?|s(?:v)?)|A(?:rray(?:ParameteriAPPLE|RangeAPPLE)|ttrib(?:1(?:d(?:ARB|v(?:ARB)?)?|f(?:ARB|v(?:ARB)?)?|s(?:ARB|v(?:ARB)?)?)|2(?:d(?:ARB|v(?:ARB)?)?|f(?:ARB|v(?:ARB)?)?|s(?:ARB|v(?:ARB)?)?)|3(?:d(?:ARB|v(?:ARB)?)?|f(?:ARB|v(?:ARB)?)?|s(?:ARB|v(?:ARB)?)?)|4(?:N(?:bv(?:ARB)?|iv(?:ARB)?|sv(?:ARB)?|u(?:b(?:ARB|v(?:ARB)?)?|iv(?:ARB)?|sv(?:ARB)?))|bv(?:ARB)?|d(?:ARB|v(?:ARB)?)?|f(?:ARB|v(?:ARB)?)?|iv(?:ARB)?|s(?:ARB|v(?:ARB)?)?|u(?:bv(?:ARB)?|iv(?:ARB)?|sv(?:ARB)?))|DivisorARB|I(?:1(?:i(?:EXT|vEXT)|ui(?:EXT|vEXT))|2(?:i(?:EXT|vEXT)|ui(?:EXT|vEXT))|3(?:i(?:EXT|vEXT)|ui(?:EXT|vEXT))|4(?:bvEXT|i(?:EXT|vEXT)|svEXT|u(?:bvEXT|i(?:EXT|vEXT)|svEXT))|PointerEXT)|Pointer(?:ARB)?))|BlendARB|Point(?:SizefAPPLE|er))|iewport)|W(?:aitSync|eight(?:PointerARB|bvARB|dvARB|fvARB|ivARB|svARB|u(?:bvARB|ivARB|svARB))|indowPos(?:2(?:d(?:ARB|v(?:ARB)?)?|f(?:ARB|v(?:ARB)?)?|i(?:ARB|v(?:ARB)?)?|s(?:ARB|v(?:ARB)?)?)|3(?:d(?:ARB|v(?:ARB)?)?|f(?:ARB|v(?:ARB)?)?|i(?:ARB|v(?:ARB)?)?|s(?:ARB|v(?:ARB)?)?)))))|host_(?:c(?:heck_multiuser_mode|reate_mach_voucher(?:_trap)?)|default_memory_manager|get_(?:UNDServer|atm_diagnostic_flag|boot_info|clock_(?:control|service)|exception_ports|io_master|multiuser_config_flags|special_port)|info|kernel_version|lockgroup_info|p(?:age_size|r(?:iv_statistics|ocessor(?:_(?:info|set(?:_priv|s))|s)))|re(?:boot|gister_(?:mach_voucher_attr_manager|well_known_mach_voucher_attr_manager)|quest_notification)|s(?:e(?:curity_(?:create_task_token|set_task_token)|t_(?:UNDServer|atm_diagnostic_flag|exception_ports|multiuser_config_flags|special_port))|tatistics(?:64)?|wap_exception_ports)|virtual_physical_table_info)|i(?:max(?:abs|div)|s(?:a(?:l(?:num|pha)|scii)|blank|cntrl|digit|graph|hexnumber|ideogram|lower|number|p(?:honogram|rint|unct)|rune|sp(?:ace|ecial)|upper|xdigit))|k(?:ext_request|mod_(?:c(?:ontrol|reate)|destroy|get_info))|l(?:dtox80|isten|ock_(?:acquire|handoff(?:_accept)?|make_stable|release|set_(?:create|destroy)|try))|m(?:a(?:c(?:h_(?:error(?:_(?:string|type))?|generate_activity_id|host_self|m(?:ake_memory_entry(?:_64)?|emory_(?:info|object_memory_entry(?:_64)?)|sg(?:_(?:destroy|overwrite|receive|se(?:nd|rver(?:_(?:importance|once))?)))?)|port(?:_(?:allocate(?:_(?:full|name|qos))?|construct|d(?:e(?:allocate|str(?:oy|uct))|nrequest_info)|extract_(?:member|right)|g(?:et_(?:attributes|context|refs|s(?:et_status|rights))|uard(?:_with_flags)?)|insert_(?:member|right)|k(?:ernel_object|object(?:_description)?)|mo(?:d_refs|ve_member)|names|peek|re(?:name|quest_notification)|s(?:et_(?:attributes|context|mscount|seqno)|pace_(?:basic_info|info)|wap_guard)|type|unguard)|s_(?:lookup|register))|thread_self|v(?:m_(?:region_info(?:_64)?|wire)|oucher_(?:deallocate|extract_attr_recipe_trap))|zone_info(?:_for_zone)?)|x_(?:backing_store_(?:recovery|suspend)|swapo(?:ff|n)|triggers))|dvise|lloc|trix_(?:multiply|scale))|i(?:g_(?:allocate|dealloc(?:_reply_port|ate)|get_reply_port|put_reply_port|reply_setup|strncpy(?:_zerofill)?)|n(?:core|herit))|lock(?:all)?|map|protect|sync|un(?:lock(?:all)?|map))|num2decl|p(?:anic(?:_init)?|fctlinput|id_for_task|osix_m(?:advise|emalign)|rocessor_(?:assign|control|exit|get_assignment|info|s(?:et_(?:create|de(?:fault|stroy)|info|max_priority|policy_(?:control|disable|enable)|sta(?:ck_usage|tistics)|t(?:asks|hreads))|tart)))|re(?:alloc|cv(?:from|msg)?|lationl)|s(?:afe_gets|e(?:c_re(?:lease|tain)|maphore_(?:create|destroy|signal(?:_(?:all|thread))?|timedwait(?:_signal)?|wait(?:_signal)?)|nd(?:file|msg|to)?|t(?:au(?:dit_addr|id)|sockopt))|h(?:m_(?:open|unlink)|utdown)|imd_(?:a(?:bs|ct|dd|l(?:l|most_equal_elements(?:_relative)?)|n(?:gle|y)|xis)|b(?:ezier|itselect)|c(?:har(?:_sat)?|lamp|onjugate|ross)|d(?:eterminant|i(?:agonal_matrix|stance(?:_squared)?)|o(?:t|uble))|equal|f(?:ast_(?:distance|length|normalize|project|r(?:ecip|sqrt))|loat|ract)|i(?:mag|n(?:circle|sphere|t(?:_sat)?|verse))|l(?:ength(?:_squared)?|inear_combination|ong(?:_sat)?)|m(?:a(?:ke_(?:char(?:16(?:_undef)?|2(?:_undef)?|3(?:2(?:_undef)?|_undef)?|4(?:_undef)?|64(?:_undef)?|8(?:_undef)?)|double(?:2(?:_undef)?|3(?:_undef)?|4(?:_undef)?|8(?:_undef)?)|float(?:16(?:_undef)?|2(?:_undef)?|3(?:_undef)?|4(?:_undef)?|8(?:_undef)?)|int(?:16(?:_undef)?|2(?:_undef)?|3(?:_undef)?|4(?:_undef)?|8(?:_undef)?)|long(?:2(?:_undef)?|3(?:_undef)?|4(?:_undef)?|8(?:_undef)?)|short(?:16(?:_undef)?|2(?:_undef)?|3(?:2(?:_undef)?|_undef)?|4(?:_undef)?|8(?:_undef)?)|u(?:char(?:16(?:_undef)?|2(?:_undef)?|3(?:2(?:_undef)?|_undef)?|4(?:_undef)?|64(?:_undef)?|8(?:_undef)?)|int(?:16(?:_undef)?|2(?:_undef)?|3(?:_undef)?|4(?:_undef)?|8(?:_undef)?)|long(?:2(?:_undef)?|3(?:_undef)?|4(?:_undef)?|8(?:_undef)?)|short(?:16(?:_undef)?|2(?:_undef)?|3(?:2(?:_undef)?|_undef)?|4(?:_undef)?|8(?:_undef)?)))|trix(?:3x3|4x4|_from_rows)?|x)|i(?:n|x)|ul)|n(?:egate|orm(?:_(?:inf|one)|alize))|orient|pr(?:ecise_(?:distance|length|normalize|project|r(?:ecip|sqrt))|oject)|quaternion|r(?:e(?:al|cip|duce_(?:add|m(?:ax|in))|f(?:lect|ract))|sqrt)|s(?:elect|hort(?:_sat)?|ign|lerp(?:_longest)?|moothstep|pline|tep|ub)|transpose|u(?:char(?:_sat)?|int(?:_sat)?|long(?:_sat)?|short(?:_sat)?))|lot_name|ock(?:atmark|et(?:pair)?)|trto(?:imax|umax)|wtch(?:_pri)?)|t(?:ask_(?:assign(?:_default)?|create(?:_suid_cred)?|for_pid|ge(?:nerate_corpse|t_(?:assignment|dyld_image_infos|e(?:mulation_vector|xc(?:_guard_behavior|eption_ports))|mach_voucher|s(?:pecial_port|tate)))|in(?:fo|spect)|map_corpse_info(?:_64)?|name_for_pid|p(?:olicy(?:_(?:get|set))?|urgable_info)|re(?:gister_dyld_(?:get_process_state|image_infos|s(?:et_dyld_state|hared_cache_image_info))|sume(?:2)?)|s(?:ample|e(?:lf_trap|t_(?:e(?:mulation(?:_vector)?|xc(?:_guard_behavior|eption_ports))|info|mach_voucher|p(?:hys_footprint_limit|o(?:licy|rt_space))|ras_pc|s(?:pecial_port|tate)))|uspend(?:2)?|wap_(?:exception_ports|mach_voucher))|t(?:erminate|hreads)|unregister_dyld_image_infos|wire|zone_info)|hread_(?:a(?:bort(?:_safely)?|ssign(?:_default)?)|create(?:_running)?|depress_abort|get_(?:assignment|exception_ports|mach_voucher|s(?:pecial_port|tate))|info|policy(?:_(?:get|set))?|resume|s(?:ample|et_(?:exception_ports|mach_voucher|policy|s(?:pecial_port|tate))|uspend|w(?:ap_(?:exception_ports|mach_voucher)|itch))|terminate|wire)|o(?:ascii|lower|upper))|uuid_(?:c(?:lear|o(?:mpare|py))|generate(?:_(?:early_random|random|time))?|is_null|parse|unparse(?:_(?:lower|upper))?)|v(?:AEBuild(?:AppleEvent|Desc|Parameters)|alloc|ector(?:16|2|3(?:2)?|4|8)|m_(?:allocate(?:_cpm)?|behavior_set|copy|deallocate|inherit|m(?:a(?:chine_attribute|p(?:_(?:64|exec_lockdown|page_query)|ped_pages_info)?)|sync)|p(?:rotect|urgable_control)|re(?:ad(?:_(?:list|overwrite))?|gion(?:_(?:64|recurse(?:_64)?))?|map)|w(?:ire|rite))|oucher_mach_msg_(?:adopt|clear|revert|set))|wcsto(?:imax|umax)|x(?:80told|pc_(?:array_(?:app(?:end_value|ly)|create(?:_connection)?|dup_fd|get_(?:bool|count|d(?:at(?:a|e)|ouble)|int64|string|u(?:int64|uid)|value)|set_(?:bool|connection|d(?:at(?:a|e)|ouble)|fd|int64|string|u(?:int64|uid)|value))|bool_(?:create|get_value)|co(?:nnection_(?:c(?:ancel|reate(?:_(?:from_endpoint|mach_service))?)|get_(?:asid|context|e(?:gid|uid)|name|pid)|resume|s(?:e(?:nd_(?:barrier|message(?:_with_reply(?:_sync)?)?)|t_(?:context|event_handler|finalizer_f|target_queue))|uspend))|py(?:_description)?)|d(?:at(?:a_(?:create(?:_with_dispatch_data)?|get_(?:bytes(?:_ptr)?|length))|e_(?:create(?:_from_current)?|get_value))|ebugger_api_misuse_info|ictionary_(?:apply|create(?:_(?:connection|reply))?|dup_fd|get_(?:bool|count|d(?:at(?:a|e)|ouble)|int64|remote_connection|string|u(?:int64|uid)|value)|set_(?:bool|connection|d(?:at(?:a|e)|ouble)|fd|int64|string|u(?:int64|uid)|value))|ouble_(?:create|get_value))|e(?:ndpoint_create|qual)|fd_(?:create|dup)|get_type|hash|int64_(?:create|get_value)|main|null_create|re(?:lease|tain)|s(?:et_event_stream_handler|hmem_(?:create|map)|tring_(?:create(?:_with_format(?:_and_arguments)?)?|get_(?:length|string_ptr)))|transaction_(?:begin|end)|u(?:int64_(?:create|get_value)|uid_(?:create|get_bytes)))))\\b)"
+ },
{
"captures": {
"1": {
@@ -376,7 +898,7 @@
"name": "support.function.cf.c"
}
},
- "match": "(\\s*)(\\bCF(?:A(?:bsoluteTimeGetCurrent|llocator(?:Allocate|Create|Deallocate|Get(?:Context|Default|PreferredSizeForSize|TypeID)|Reallocate|SetDefault)|rray(?:App(?:end(?:Array|Value)|lyFunction)|BSearchValues|C(?:ontainsValue|reate(?:Copy|Mutable(?:Copy)?)?)|ExchangeValuesAtIndices|Get(?:Count(?:OfValue)?|FirstIndexOfValue|LastIndexOfValue|TypeID|Value(?:AtIndex|s))|InsertValueAtIndex|Re(?:move(?:AllValues|ValueAtIndex)|placeValues)|S(?:etValueAtIndex|ortValues))|ttributedString(?:BeginEditing|Create(?:Copy|Mutable(?:Copy)?|WithSubstring)?|EndEditing|Get(?:Attribute(?:AndLongestEffectiveRange|s(?:AndLongestEffectiveRange)?)?|Length|MutableString|String|TypeID)|Re(?:moveAttribute|place(?:AttributedString|String))|SetAttribute(?:s)?))|B(?:ag(?:A(?:ddValue|pplyFunction)|C(?:ontainsValue|reate(?:Copy|Mutable(?:Copy)?)?)|Get(?:Count(?:OfValue)?|TypeID|Value(?:IfPresent|s)?)|Re(?:move(?:AllValues|Value)|placeValue)|SetValue)|i(?:naryHeap(?:A(?:ddValue|pplyFunction)|C(?:ontainsValue|reate(?:Copy)?)|Get(?:Count(?:OfValue)?|Minimum(?:IfPresent)?|TypeID|Values)|Remove(?:AllValues|MinimumValue))|tVector(?:C(?:ontainsBit|reate(?:Copy|Mutable(?:Copy)?)?)|FlipBit(?:AtIndex|s)|Get(?:Bit(?:AtIndex|s)|Count(?:OfBit)?|FirstIndexOfBit|LastIndexOfBit|TypeID)|Set(?:AllBits|Bit(?:AtIndex|s)|Count)))|ooleanGet(?:TypeID|Value)|undle(?:C(?:loseBundleResourceMap|opy(?:AuxiliaryExecutableURL|Bu(?:iltInPlugInsURL|ndle(?:Localizations|URL))|Executable(?:Architectures(?:ForURL)?|URL)|InfoDictionary(?:ForURL|InDirectory)|Localiz(?:ationsFor(?:Preferences|URL)|edString)|Pr(?:eferredLocalizationsFromArray|ivateFrameworksURL)|Resource(?:URL(?:ForLocalization|InDirectory|sOfType(?:ForLocalization|InDirectory)?)?|sDirectoryURL)|S(?:hared(?:FrameworksURL|SupportURL)|upportFilesDirectoryURL))|reate(?:BundlesFromDirectory)?)|Get(?:AllBundles|BundleWithIdentifier|D(?:ataPointer(?:ForName|sForNames)|evelopmentRegion)|FunctionPointer(?:ForName|sForNames)|I(?:dentifier|nfoDictionary)|LocalInfoDictionary|MainBundle|P(?:ackageInfo(?:InDirectory)?|lugIn)|TypeID|V(?:alueForInfoDictionaryKey|ersionNumber))|IsExecutableLoaded|LoadExecutable(?:AndReturnError)?|OpenBundleResource(?:Files|Map)|PreflightExecutable|UnloadExecutable)|yteOrderGetCurrent)|C(?:alendar(?:AddComponents|C(?:o(?:mposeAbsoluteTime|py(?:Current|Locale|TimeZone))|reateWithIdentifier)|DecomposeAbsoluteTime|Get(?:ComponentDifference|FirstWeekday|Identifier|M(?:aximumRangeOfUnit|inimum(?:DaysInFirstWeek|RangeOfUnit))|OrdinalityOfUnit|RangeOfUnit|T(?:imeRangeOfUnit|ypeID))|Set(?:FirstWeekday|Locale|MinimumDaysInFirstWeek|TimeZone))|haracterSet(?:AddCharactersIn(?:Range|String)|Create(?:BitmapRepresentation|Copy|InvertedSet|Mutable(?:Copy)?|With(?:BitmapRepresentation|CharactersIn(?:Range|String)))|Get(?:Predefined|TypeID)|HasMemberInPlane|I(?:n(?:tersect|vert)|s(?:CharacterMember|LongCharacterMember|SupersetOfSet))|RemoveCharactersIn(?:Range|String)|Union)|o(?:nvert(?:Double(?:HostToSwapped|SwappedToHost)|Float(?:32(?:HostToSwapped|SwappedToHost)|64(?:HostToSwapped|SwappedToHost)|HostToSwapped|SwappedToHost))|py(?:Description|HomeDirectoryURL|TypeIDDescription)))|D(?:at(?:a(?:AppendBytes|Create(?:Copy|Mutable(?:Copy)?|WithBytesNoCopy)?|DeleteBytes|Find|Get(?:Byte(?:Ptr|s)|Length|MutableBytePtr|TypeID)|IncreaseLength|ReplaceBytes|SetLength)|e(?:C(?:ompare|reate)|Formatter(?:C(?:opyProperty|reate(?:DateF(?:ormatFromTemplate|romString)|StringWith(?:AbsoluteTime|Date))?)|Get(?:AbsoluteTimeFromString|DateStyle|Format|Locale|T(?:imeStyle|ypeID))|Set(?:Format|Property))|Get(?:AbsoluteTime|T(?:imeIntervalSinceDate|ypeID))))|ictionary(?:A(?:ddValue|pplyFunction)|C(?:ontains(?:Key|Value)|reate(?:Copy|Mutable(?:Copy)?)?)|Get(?:Count(?:Of(?:Key|Value))?|KeysAndValues|TypeID|Value(?:IfPresent)?)|Re(?:move(?:AllValues|Value)|placeValue)|SetValue))|E(?:qual|rror(?:C(?:opy(?:Description|FailureReason|RecoverySuggestion|UserInfo)|reate(?:WithUserInfoKeysAndValues)?)|Get(?:Code|Domain|TypeID)))|File(?:Descriptor(?:Create(?:RunLoopSource)?|DisableCallBacks|EnableCallBacks|Get(?:Context|NativeDescriptor|TypeID)|I(?:nvalidate|sValid))|Security(?:C(?:opy(?:AccessControlList|GroupUUID|OwnerUUID)|reate(?:Copy)?)|Get(?:Group|Mode|Owner|TypeID)|Set(?:AccessControlList|Group(?:UUID)?|Mode|Owner(?:UUID)?)))|Get(?:Allocator|RetainCount|TypeID)|Hash|Locale(?:C(?:opy(?:AvailableLocaleIdentifiers|C(?:ommonISOCurrencyCodes|urrent)|DisplayNameForPropertyValue|ISO(?:C(?:ountryCodes|urrencyCodes)|LanguageCodes)|PreferredLanguages)|reate(?:C(?:anonicalL(?:anguageIdentifierFromString|ocaleIdentifierFromS(?:criptManagerCodes|tring))|o(?:mponentsFromLocaleIdentifier|py))|LocaleIdentifierFrom(?:Components|WindowsLocaleCode))?)|Get(?:Identifier|Language(?:CharacterDirection|LineDirection)|System|TypeID|Value|WindowsLocaleCodeFromLocaleIdentifier))|M(?:a(?:chPort(?:Create(?:RunLoopSource|WithPort)?|Get(?:Context|InvalidationCallBack|Port|TypeID)|I(?:nvalidate|sValid)|SetInvalidationCallBack)|keCollectable)|essagePort(?:Create(?:Local|R(?:emote|unLoopSource))|Get(?:Context|InvalidationCallBack|Name|TypeID)|I(?:nvalidate|s(?:Remote|Valid))|Se(?:ndRequest|t(?:DispatchQueue|InvalidationCallBack|Name))))|N(?:otificationCenter(?:AddObserver|Get(?:D(?:arwinNotifyCenter|istributedCenter)|LocalCenter|TypeID)|PostNotification(?:WithOptions)?|Remove(?:EveryObserver|Observer))|u(?:llGetTypeID|mber(?:C(?:ompare|reate)|Formatter(?:C(?:opyProperty|reate(?:NumberFromString|StringWith(?:Number|Value))?)|Get(?:DecimalInfoForCurrencyCode|Format|Locale|Style|TypeID|ValueFromString)|Set(?:Format|Property))|Get(?:ByteSize|Type(?:ID)?|Value)|IsFloatType)))|P(?:lugIn(?:AddInstanceForFactory|Create|FindFactoriesForPlugInType(?:InPlugIn)?|Get(?:Bundle|TypeID)|I(?:nstance(?:Create(?:WithInstanceDataSize)?|Get(?:FactoryName|In(?:stanceData|terfaceFunctionTable)|TypeID))|sLoadOnDemand)|Re(?:gister(?:FactoryFunction(?:ByName)?|PlugInType)|moveInstanceForFactory)|SetLoadOnDemand|Unregister(?:Factory|PlugInType))|r(?:eferences(?:A(?:ddSuitePreferencesToApp|pp(?:Synchronize|ValueIsForced))|Copy(?:AppValue|KeyList|Multiple|Value)|GetApp(?:BooleanValue|IntegerValue)|RemoveSuitePreferencesFromApp|S(?:et(?:AppValue|Multiple|Value)|ynchronize))|opertyList(?:Create(?:D(?:ata|eepCopy)|With(?:Data|Stream))|IsValid|Write)))|R(?:angeMake|e(?:adStream(?:C(?:lose|opy(?:Error|Property)|reateWith(?:BytesNoCopy|File))|Get(?:Buffer|Error|Status|TypeID)|HasBytesAvailable|Open|Read|S(?:cheduleWithRunLoop|et(?:Client|Property))|UnscheduleFromRunLoop)|lease|tain)|unLoop(?:Add(?:CommonMode|Observer|Source|Timer)|Co(?:ntains(?:Observer|Source|Timer)|py(?:AllModes|CurrentMode))|Get(?:Current|Main|NextTimerFireDate|TypeID)|IsWaiting|Observer(?:Create(?:WithHandler)?|DoesRepeat|Get(?:Activities|Context|Order|TypeID)|I(?:nvalidate|sValid))|PerformBlock|R(?:emove(?:Observer|Source|Timer)|un(?:InMode)?)|S(?:ource(?:Create|Get(?:Context|Order|TypeID)|I(?:nvalidate|sValid)|Signal)|top)|Timer(?:Create(?:WithHandler)?|DoesRepeat|Get(?:Context|Interval|NextFireDate|Order|TypeID)|I(?:nvalidate|sValid)|SetNextFireDate)|WakeUp))|S(?:et(?:A(?:ddValue|pplyFunction)|C(?:ontainsValue|reate(?:Copy|Mutable(?:Copy)?)?)|Get(?:Count(?:OfValue)?|TypeID|Value(?:IfPresent|s)?)|Re(?:move(?:AllValues|Value)|placeValue)|SetValue)|how(?:Str)?|ocket(?:C(?:o(?:nnectToAddress|py(?:Address|PeerAddress|Registered(?:SocketSignature|Value)))|reate(?:ConnectedToSocketSignature|RunLoopSource|With(?:Native|SocketSignature))?)|DisableCallBacks|EnableCallBacks|Get(?:Context|DefaultNameRegistryPortNumber|Native|SocketFlags|TypeID)|I(?:nvalidate|sValid)|Register(?:SocketSignature|Value)|Se(?:ndData|t(?:Address|DefaultNameRegistryPortNumber|SocketFlags))|Unregister)|tr(?:eamCreate(?:BoundPair|PairWith(?:PeerSocketSignature|Socket(?:ToHost)?))|ing(?:Append(?:C(?:String|haracters)|Format(?:AndArguments)?|PascalString)?|C(?:apitalize|o(?:mpare(?:WithOptions(?:AndLocale)?)?|nvert(?:EncodingTo(?:IANACharSetName|NSStringEncoding|WindowsCodepage)|IANACharSetNameToEncoding|NSStringEncodingToEncoding|WindowsCodepageToEncoding))|reate(?:Array(?:BySeparatingStrings|WithFindResults)|ByCombiningStrings|Copy|ExternalRepresentation|FromExternalRepresentation|Mutable(?:Copy|WithExternalCharactersNoCopy)?|With(?:Bytes(?:NoCopy)?|C(?:String(?:NoCopy)?|haracters(?:NoCopy)?)|F(?:ileSystemRepresentation|ormat(?:AndArguments)?)|PascalString(?:NoCopy)?|Substring)))|Delete|F(?:ind(?:AndReplace|CharacterFromSet|WithOptions(?:AndLocale)?)?|old)|Get(?:Bytes|C(?:String(?:Ptr)?|haracter(?:AtIndex|FromInlineBuffer|s(?:Ptr)?))|DoubleValue|F(?:astestEncoding|ileSystemRepresentation)|HyphenationLocationBeforeIndex|IntValue|L(?:ength|i(?:neBounds|stOfAvailableEncodings)|ongCharacterForSurrogatePair)|M(?:aximumSize(?:ForEncoding|OfFileSystemRepresentation)|ostCompatibleMacStringEncoding)|NameOfEncoding|Pa(?:ragraphBounds|scalString(?:Ptr)?)|RangeOfComposedCharactersAtIndex|S(?:mallestEncoding|urrogatePairForLongCharacter|ystemEncoding)|TypeID)|Has(?:Prefix|Suffix)|I(?:n(?:itInlineBuffer|sert)|s(?:EncodingAvailable|HyphenationAvailableForLocale|Surrogate(?:HighCharacter|LowCharacter)))|Lowercase|Normalize|Pad|Replace(?:All)?|SetExternalCharactersNoCopy|T(?:okenizer(?:AdvanceToNextToken|C(?:opy(?:BestStringLanguage|CurrentTokenAttribute)|reate)|G(?:et(?:Current(?:SubTokens|TokenRange)|TypeID)|oToTokenAtIndex)|SetString)|r(?:ansform|im(?:Whitespace)?))|Uppercase))|wapInt(?:16(?:BigToHost|HostTo(?:Big|Little)|LittleToHost)?|32(?:BigToHost|HostTo(?:Big|Little)|LittleToHost)?|64(?:BigToHost|HostTo(?:Big|Little)|LittleToHost)?))|T(?:imeZone(?:C(?:opy(?:Abbreviation(?:Dictionary)?|Default|KnownNames|LocalizedName|System)|reate(?:With(?:Name|TimeIntervalFromGMT))?)|Get(?:Da(?:ta|ylightSavingTimeOffset)|N(?:ame|extDaylightSavingTimeTransition)|SecondsFromGMT|TypeID)|IsDaylightSavingTime|ResetSystem|Set(?:AbbreviationDictionary|Default))|ree(?:App(?:endChild|lyFunctionToChildren)|Create|FindRoot|Get(?:C(?:hild(?:AtIndex|Count|ren)|ontext)|FirstChild|NextSibling|Parent|TypeID)|InsertSibling|PrependChild|Remove(?:AllChildren)?|S(?:etContext|ortChildren)))|U(?:RL(?:C(?:anBeDecomposed|learResourcePropertyCache(?:ForKey)?|opy(?:AbsoluteURL|F(?:ileSystemPath|ragment)|HostName|LastPathComponent|NetLocation|Pa(?:rameterString|ssword|th(?:Extension)?)|QueryString|Resource(?:Propert(?:iesForKeys|yForKey)|Specifier)|S(?:cheme|trictPath)|UserName)|reate(?:AbsoluteURLWithBytes|B(?:ookmarkData(?:From(?:AliasRecord|File))?|yResolvingBookmarkData)|Copy(?:AppendingPath(?:Component|Extension)|Deleting(?:LastPathComponent|PathExtension))|Data|F(?:ile(?:PathURL|ReferenceURL)|romFileSystemRepresentation(?:RelativeToBase)?)|ResourcePropert(?:iesForKeysFromBookmarkData|yForKeyFromBookmarkData)|StringByReplacingPercentEscapes|With(?:Bytes|FileSystemPath(?:RelativeToBase)?|String)))|Enumerator(?:CreateFor(?:DirectoryURL|MountedVolumes)|Get(?:DescendentLevel|NextURL|TypeID)|SkipDescendents)|Get(?:B(?:aseURL|yte(?:RangeForComponent|s))|FileSystemRepresentation|PortNumber|String|TypeID)|HasDirectoryPath|ResourceIsReachable|S(?:et(?:ResourcePropert(?:iesForKeys|yForKey)|TemporaryResourcePropertyForKey)|t(?:artAccessingSecurityScopedResource|opAccessingSecurityScopedResource))|WriteBookmarkDataToFile)|UID(?:Create(?:From(?:String|UUIDBytes)|String|WithBytes)?|Get(?:ConstantUUIDWithBytes|TypeID|UUIDBytes))|serNotification(?:C(?:ancel|heckBoxChecked|reate(?:RunLoopSource)?)|Display(?:Alert|Notice)|Get(?:Response(?:Dictionary|Value)|TypeID)|PopUpSelection|ReceiveResponse|SecureTextField|Update))|WriteStream(?:C(?:anAcceptBytes|lose|opy(?:Error|Property)|reateWith(?:AllocatedBuffers|Buffer|File))|Get(?:Error|Status|TypeID)|Open|S(?:cheduleWithRunLoop|et(?:Client|Property))|UnscheduleFromRunLoop|Write)|XMLCreateStringBy(?:EscapingEntities|UnescapingEntities))\\b)"
+ "match": "(\\s*)(\\bCF(?:A(?:bsoluteTimeGetCurrent|llocator(?:Allocate|Create|Deallocate|Get(?:Context|Default|PreferredSizeForSize|TypeID)|Reallocate|SetDefault)|rray(?:App(?:end(?:Array|Value)|lyFunction)|BSearchValues|C(?:ontainsValue|reate(?:Copy|Mutable(?:Copy)?)?)|ExchangeValuesAtIndices|Get(?:Count(?:OfValue)?|FirstIndexOfValue|LastIndexOfValue|TypeID|Value(?:AtIndex|s))|InsertValueAtIndex|Re(?:move(?:AllValues|ValueAtIndex)|placeValues)|S(?:etValueAtIndex|ortValues))|ttributedString(?:BeginEditing|Create(?:Copy|Mutable(?:Copy)?|WithSubstring)?|EndEditing|Get(?:Attribute(?:AndLongestEffectiveRange|s(?:AndLongestEffectiveRange)?)?|Length|MutableString|String|TypeID)|Re(?:moveAttribute|place(?:AttributedString|String))|SetAttribute(?:s)?))|B(?:ag(?:A(?:ddValue|pplyFunction)|C(?:ontainsValue|reate(?:Copy|Mutable(?:Copy)?)?)|Get(?:Count(?:OfValue)?|TypeID|Value(?:IfPresent|s)?)|Re(?:move(?:AllValues|Value)|placeValue)|SetValue)|i(?:naryHeap(?:A(?:ddValue|pplyFunction)|C(?:ontainsValue|reate(?:Copy)?)|Get(?:Count(?:OfValue)?|Minimum(?:IfPresent)?|TypeID|Values)|Remove(?:AllValues|MinimumValue))|tVector(?:C(?:ontainsBit|reate(?:Copy|Mutable(?:Copy)?)?)|FlipBit(?:AtIndex|s)|Get(?:Bit(?:AtIndex|s)|Count(?:OfBit)?|FirstIndexOfBit|LastIndexOfBit|TypeID)|Set(?:AllBits|Bit(?:AtIndex|s)|Count)))|ooleanGet(?:TypeID|Value)|undle(?:C(?:opy(?:AuxiliaryExecutableURL|Bu(?:iltInPlugInsURL|ndle(?:Localizations|URL))|Executable(?:Architectures(?:ForURL)?|URL)|InfoDictionary(?:ForURL|InDirectory)|Localiz(?:ationsFor(?:Preferences|URL)|edString)|Pr(?:eferredLocalizationsFromArray|ivateFrameworksURL)|Resource(?:URL(?:ForLocalization|InDirectory|sOfType(?:ForLocalization|InDirectory)?)?|sDirectoryURL)|S(?:hared(?:FrameworksURL|SupportURL)|upportFilesDirectoryURL))|reate(?:BundlesFromDirectory)?)|Get(?:AllBundles|BundleWithIdentifier|D(?:ataPointer(?:ForName|sForNames)|evelopmentRegion)|FunctionPointer(?:ForName|sForNames)|I(?:dentifier|nfoDictionary)|LocalInfoDictionary|MainBundle|P(?:ackageInfo(?:InDirectory)?|lugIn)|TypeID|V(?:alueForInfoDictionaryKey|ersionNumber))|IsExecutableLoaded|LoadExecutable(?:AndReturnError)?|PreflightExecutable|UnloadExecutable)|yteOrderGetCurrent)|C(?:alendar(?:AddComponents|C(?:o(?:mposeAbsoluteTime|py(?:Current|Locale|TimeZone))|reateWithIdentifier)|DecomposeAbsoluteTime|Get(?:ComponentDifference|FirstWeekday|Identifier|M(?:aximumRangeOfUnit|inimum(?:DaysInFirstWeek|RangeOfUnit))|OrdinalityOfUnit|RangeOfUnit|T(?:imeRangeOfUnit|ypeID))|Set(?:FirstWeekday|Locale|MinimumDaysInFirstWeek|TimeZone))|haracterSet(?:AddCharactersIn(?:Range|String)|Create(?:BitmapRepresentation|Copy|InvertedSet|Mutable(?:Copy)?|With(?:BitmapRepresentation|CharactersIn(?:Range|String)))|Get(?:Predefined|TypeID)|HasMemberInPlane|I(?:n(?:tersect|vert)|s(?:CharacterMember|LongCharacterMember|SupersetOfSet))|RemoveCharactersIn(?:Range|String)|Union)|o(?:nvert(?:Double(?:HostToSwapped|SwappedToHost)|Float(?:32(?:HostToSwapped|SwappedToHost)|64(?:HostToSwapped|SwappedToHost)|HostToSwapped|SwappedToHost))|py(?:Description|HomeDirectoryURL|TypeIDDescription)))|D(?:at(?:a(?:AppendBytes|Create(?:Copy|Mutable(?:Copy)?|WithBytesNoCopy)?|DeleteBytes|Find|Get(?:Byte(?:Ptr|s)|Length|MutableBytePtr|TypeID)|IncreaseLength|ReplaceBytes|SetLength)|e(?:C(?:ompare|reate)|Formatter(?:C(?:opyProperty|reate(?:DateF(?:ormatFromTemplate|romString)|StringWith(?:AbsoluteTime|Date))?)|Get(?:AbsoluteTimeFromString|DateStyle|Format|Locale|T(?:imeStyle|ypeID))|Set(?:Format|Property))|Get(?:AbsoluteTime|T(?:imeIntervalSinceDate|ypeID))))|ictionary(?:A(?:ddValue|pplyFunction)|C(?:ontains(?:Key|Value)|reate(?:Copy|Mutable(?:Copy)?)?)|Get(?:Count(?:Of(?:Key|Value))?|KeysAndValues|TypeID|Value(?:IfPresent)?)|Re(?:move(?:AllValues|Value)|placeValue)|SetValue))|E(?:qual|rror(?:C(?:opy(?:Description|FailureReason|RecoverySuggestion|UserInfo)|reate(?:WithUserInfoKeysAndValues)?)|Get(?:Code|Domain|TypeID)))|File(?:Descriptor(?:Create(?:RunLoopSource)?|DisableCallBacks|EnableCallBacks|Get(?:Context|NativeDescriptor|TypeID)|I(?:nvalidate|sValid))|Security(?:C(?:opy(?:AccessControlList|GroupUUID|OwnerUUID)|reate(?:Copy)?)|Get(?:Group|Mode|Owner|TypeID)|Set(?:AccessControlList|Group(?:UUID)?|Mode|Owner(?:UUID)?)))|Get(?:Allocator|RetainCount|TypeID)|Hash|Locale(?:C(?:opy(?:AvailableLocaleIdentifiers|C(?:ommonISOCurrencyCodes|urrent)|DisplayNameForPropertyValue|ISO(?:C(?:ountryCodes|urrencyCodes)|LanguageCodes)|PreferredLanguages)|reate(?:C(?:anonicalL(?:anguageIdentifierFromString|ocaleIdentifierFromS(?:criptManagerCodes|tring))|o(?:mponentsFromLocaleIdentifier|py))|LocaleIdentifierFrom(?:Components|WindowsLocaleCode))?)|Get(?:Identifier|Language(?:CharacterDirection|LineDirection)|System|TypeID|Value|WindowsLocaleCodeFromLocaleIdentifier))|M(?:a(?:chPort(?:Create(?:RunLoopSource|WithPort)?|Get(?:Context|InvalidationCallBack|Port|TypeID)|I(?:nvalidate|sValid)|SetInvalidationCallBack)|keCollectable)|essagePort(?:Create(?:Local|R(?:emote|unLoopSource))|Get(?:Context|InvalidationCallBack|Name|TypeID)|I(?:nvalidate|s(?:Remote|Valid))|Se(?:ndRequest|t(?:DispatchQueue|InvalidationCallBack|Name))))|N(?:otificationCenter(?:AddObserver|Get(?:D(?:arwinNotifyCenter|istributedCenter)|LocalCenter|TypeID)|PostNotification(?:WithOptions)?|Remove(?:EveryObserver|Observer))|u(?:llGetTypeID|mber(?:C(?:ompare|reate)|Formatter(?:C(?:opyProperty|reate(?:NumberFromString|StringWith(?:Number|Value))?)|Get(?:DecimalInfoForCurrencyCode|Format|Locale|Style|TypeID|ValueFromString)|Set(?:Format|Property))|Get(?:ByteSize|Type(?:ID)?|Value)|IsFloatType)))|P(?:lugIn(?:AddInstanceForFactory|Create|FindFactoriesForPlugInType(?:InPlugIn)?|Get(?:Bundle|TypeID)|I(?:nstance(?:Create(?:WithInstanceDataSize)?|Get(?:FactoryName|In(?:stanceData|terfaceFunctionTable)|TypeID))|sLoadOnDemand)|Re(?:gister(?:FactoryFunction(?:ByName)?|PlugInType)|moveInstanceForFactory)|SetLoadOnDemand|Unregister(?:Factory|PlugInType))|r(?:eferences(?:A(?:ddSuitePreferencesToApp|pp(?:Synchronize|ValueIsForced))|Copy(?:AppValue|KeyList|Multiple|Value)|GetApp(?:BooleanValue|IntegerValue)|RemoveSuitePreferencesFromApp|S(?:et(?:AppValue|Multiple|Value)|ynchronize))|opertyList(?:Create(?:D(?:ata|eepCopy)|With(?:Data|Stream))|IsValid|Write)))|R(?:angeMake|e(?:adStream(?:C(?:lose|opy(?:Error|Property)|reateWith(?:BytesNoCopy|File))|Get(?:Buffer|Error|Status|TypeID)|HasBytesAvailable|Open|Read|S(?:cheduleWithRunLoop|et(?:Client|Property))|UnscheduleFromRunLoop)|lease|tain)|unLoop(?:Add(?:CommonMode|Observer|Source|Timer)|Co(?:ntains(?:Observer|Source|Timer)|py(?:AllModes|CurrentMode))|Get(?:Current|Main|NextTimerFireDate|TypeID)|IsWaiting|Observer(?:Create(?:WithHandler)?|DoesRepeat|Get(?:Activities|Context|Order|TypeID)|I(?:nvalidate|sValid))|PerformBlock|R(?:emove(?:Observer|Source|Timer)|un(?:InMode)?)|S(?:ource(?:Create|Get(?:Context|Order|TypeID)|I(?:nvalidate|sValid)|Signal)|top)|Timer(?:Create(?:WithHandler)?|DoesRepeat|Get(?:Context|Interval|NextFireDate|Order|TypeID)|I(?:nvalidate|sValid)|SetNextFireDate)|WakeUp))|S(?:et(?:A(?:ddValue|pplyFunction)|C(?:ontainsValue|reate(?:Copy|Mutable(?:Copy)?)?)|Get(?:Count(?:OfValue)?|TypeID|Value(?:IfPresent|s)?)|Re(?:move(?:AllValues|Value)|placeValue)|SetValue)|how(?:Str)?|ocket(?:C(?:o(?:nnectToAddress|py(?:Address|PeerAddress|Registered(?:SocketSignature|Value)))|reate(?:ConnectedToSocketSignature|RunLoopSource|With(?:Native|SocketSignature))?)|DisableCallBacks|EnableCallBacks|Get(?:Context|DefaultNameRegistryPortNumber|Native|SocketFlags|TypeID)|I(?:nvalidate|sValid)|Register(?:SocketSignature|Value)|Se(?:ndData|t(?:Address|DefaultNameRegistryPortNumber|SocketFlags))|Unregister)|tr(?:eamCreate(?:BoundPair|PairWith(?:PeerSocketSignature|Socket(?:ToHost)?))|ing(?:Append(?:C(?:String|haracters)|Format(?:AndArguments)?|PascalString)?|C(?:apitalize|o(?:mpare(?:WithOptions(?:AndLocale)?)?|nvert(?:EncodingTo(?:IANACharSetName|NSStringEncoding|WindowsCodepage)|IANACharSetNameToEncoding|NSStringEncodingToEncoding|WindowsCodepageToEncoding))|reate(?:Array(?:BySeparatingStrings|WithFindResults)|ByCombiningStrings|Copy|ExternalRepresentation|FromExternalRepresentation|Mutable(?:Copy|WithExternalCharactersNoCopy)?|With(?:Bytes(?:NoCopy)?|C(?:String(?:NoCopy)?|haracters(?:NoCopy)?)|F(?:ileSystemRepresentation|ormat(?:AndArguments)?)|PascalString(?:NoCopy)?|Substring)))|Delete|F(?:ind(?:AndReplace|CharacterFromSet|WithOptions(?:AndLocale)?)?|old)|Get(?:Bytes|C(?:String(?:Ptr)?|haracter(?:AtIndex|FromInlineBuffer|s(?:Ptr)?))|DoubleValue|F(?:astestEncoding|ileSystemRepresentation)|HyphenationLocationBeforeIndex|IntValue|L(?:ength|i(?:neBounds|stOfAvailableEncodings)|ongCharacterForSurrogatePair)|M(?:aximumSize(?:ForEncoding|OfFileSystemRepresentation)|ostCompatibleMacStringEncoding)|NameOfEncoding|Pa(?:ragraphBounds|scalString(?:Ptr)?)|RangeOfComposedCharactersAtIndex|S(?:mallestEncoding|urrogatePairForLongCharacter|ystemEncoding)|TypeID)|Has(?:Prefix|Suffix)|I(?:n(?:itInlineBuffer|sert)|s(?:EncodingAvailable|HyphenationAvailableForLocale|Surrogate(?:HighCharacter|LowCharacter)))|Lowercase|Normalize|Pad|Replace(?:All)?|SetExternalCharactersNoCopy|T(?:okenizer(?:AdvanceToNextToken|C(?:opy(?:BestStringLanguage|CurrentTokenAttribute)|reate)|G(?:et(?:Current(?:SubTokens|TokenRange)|TypeID)|oToTokenAtIndex)|SetString)|r(?:ansform|im(?:Whitespace)?))|Uppercase))|wapInt(?:16(?:BigToHost|HostTo(?:Big|Little)|LittleToHost)?|32(?:BigToHost|HostTo(?:Big|Little)|LittleToHost)?|64(?:BigToHost|HostTo(?:Big|Little)|LittleToHost)?))|T(?:imeZone(?:C(?:opy(?:Abbreviation(?:Dictionary)?|Default|KnownNames|LocalizedName|System)|reate(?:With(?:Name|TimeIntervalFromGMT))?)|Get(?:Da(?:ta|ylightSavingTimeOffset)|N(?:ame|extDaylightSavingTimeTransition)|SecondsFromGMT|TypeID)|IsDaylightSavingTime|ResetSystem|Set(?:AbbreviationDictionary|Default))|ree(?:App(?:endChild|lyFunctionToChildren)|Create|FindRoot|Get(?:C(?:hild(?:AtIndex|Count|ren)|ontext)|FirstChild|NextSibling|Parent|TypeID)|InsertSibling|PrependChild|Remove(?:AllChildren)?|S(?:etContext|ortChildren)))|U(?:RL(?:C(?:anBeDecomposed|learResourcePropertyCache(?:ForKey)?|opy(?:AbsoluteURL|F(?:ileSystemPath|ragment)|HostName|LastPathComponent|NetLocation|Pa(?:ssword|th(?:Extension)?)|QueryString|Resource(?:Propert(?:iesForKeys|yForKey)|Specifier)|S(?:cheme|trictPath)|UserName)|reate(?:AbsoluteURLWithBytes|B(?:ookmarkData(?:From(?:AliasRecord|File))?|yResolvingBookmarkData)|Copy(?:AppendingPath(?:Component|Extension)|Deleting(?:LastPathComponent|PathExtension))|Data|F(?:ile(?:PathURL|ReferenceURL)|romFileSystemRepresentation(?:RelativeToBase)?)|ResourcePropert(?:iesForKeysFromBookmarkData|yForKeyFromBookmarkData)|StringByReplacingPercentEscapes|With(?:Bytes|FileSystemPath(?:RelativeToBase)?|String)))|Enumerator(?:CreateFor(?:DirectoryURL|MountedVolumes)|Get(?:DescendentLevel|NextURL|TypeID)|SkipDescendents)|Get(?:B(?:aseURL|yte(?:RangeForComponent|s))|FileSystemRepresentation|PortNumber|String|TypeID)|HasDirectoryPath|ResourceIsReachable|S(?:et(?:ResourcePropert(?:iesForKeys|yForKey)|TemporaryResourcePropertyForKey)|t(?:artAccessingSecurityScopedResource|opAccessingSecurityScopedResource))|WriteBookmarkDataToFile)|UID(?:Create(?:From(?:String|UUIDBytes)|String|WithBytes)?|Get(?:ConstantUUIDWithBytes|TypeID|UUIDBytes))|serNotification(?:C(?:ancel|heckBoxChecked|reate(?:RunLoopSource)?)|Display(?:Alert|Notice)|Get(?:Response(?:Dictionary|Value)|TypeID)|PopUpSelection|ReceiveResponse|SecureTextField|Update))|WriteStream(?:C(?:anAcceptBytes|lose|opy(?:Error|Property)|reateWith(?:AllocatedBuffers|Buffer|File))|Get(?:Error|Status|TypeID)|Open|S(?:cheduleWithRunLoop|et(?:Client|Property))|UnscheduleFromRunLoop|Write)|XMLCreateStringBy(?:EscapingEntities|UnescapingEntities))\\b)"
},
{
"captures": {
@@ -400,6 +922,28 @@
},
"match": "(\\s*)(\\b(?:clock_(?:get(?:res|time(?:_nsec_np)?)|settime)|mk(?:ostemp(?:s)?|pathat_np)|rename(?:atx_np|x_np)|timingsafe_bcmp)\\b)"
},
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "support.function.clib.10.13.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:fmemopen|mk(?:dtempat_np|ostempsat_np|stempsat_np)|open_memstream|ptsname_r|setattrlistat)\\b)"
+ },
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "support.function.clib.10.15.c"
+ }
+ },
+ "match": "(\\s*)(\\b(?:rpmatch|timespec_get)\\b)"
+ },
{
"captures": {
"1": {
@@ -420,7 +964,7 @@
"name": "support.function.clib.10.9.c"
}
},
- "match": "(\\s*)(\\bf(?:fsll|lsll)\\b)"
+ "match": "(\\s*)(\\b(?:f(?:fsll|lsll)|memset_s)\\b)"
},
{
"captures": {
@@ -431,7 +975,7 @@
"name": "support.function.clib.c"
}
},
- "match": "(\\s*)(\\b(?:a(?:64l|b(?:ort|s)|c(?:c(?:ess(?:x_np)?|t)|os(?:f|h(?:f|l)?|l)?)|dd_profil|l(?:arm|loca)|rc4random(?:_(?:addrandom|buf|stir|uniform))?|s(?:ctime(?:_r)?|in(?:f|h(?:f|l)?|l)?|printf)|t(?:an(?:2(?:f|l)?|f|h(?:f|l)?|l)?|exit(?:_b)?|o(?:f|i|l(?:l)?)))|b(?:c(?:mp|opy)|rk|s(?:d_signal|earch(?:_b)?)|zero)|c(?:alloc|brt(?:f|l)?|eil(?:f|l)?|get(?:c(?:ap|lose)|ent|first|match|n(?:ext|um)|s(?:et|tr)|ustr)|h(?:dir|own|root)|l(?:earerr|o(?:ck|se))|o(?:nfstr|pysign(?:f|l)?|s(?:f|h(?:f|l)?|l)?)|r(?:eat|ypt)|t(?:ermid(?:_r)?|ime(?:_r)?))|d(?:evname(?:_r)?|i(?:fftime|gittoint|spatch_(?:time|walltime)|v)|printf|rand48|up(?:2)?)|e(?:cvt|n(?:crypt|dusershell)|r(?:and48|f(?:c(?:f|l)?|f|l)?)|x(?:changedata|ec(?:l(?:e|p)?|v(?:P|e|p)?)|it|p(?:2(?:f|l)?|f|l|m1(?:f|l)?)?))|f(?:abs(?:f|l)?|c(?:h(?:dir|own)|lose|ntl|vt)|d(?:im(?:f|l)?|open)|e(?:of|rror)|f(?:l(?:agstostr|ush)|s(?:ctl|l)?)|get(?:attrlist|c|ln|pos|s)|ile(?:no|sec_(?:dup|free|get_property|init|query_property|set_property|unset_property))|l(?:o(?:ck(?:file)?|or(?:f|l)?)|s(?:l)?)|m(?:a(?:f|l|x(?:f|l)?)?|in(?:f|l)?|od(?:f|l)?|tcheck)|o(?:pen|rk)|p(?:athconf|rintf|u(?:rge|t(?:c|s)))|re(?:ad|e|open|xp(?:f|l)?)|s(?:c(?:anf|tl)|e(?:ek(?:o)?|t(?:attrlist|pos))|ync)|t(?:ell(?:o)?|r(?:uncate|ylockfile))|un(?:lockfile|open)|write)|g(?:cvt|et(?:attrlist|bsize|c(?:_unlocked|har(?:_unlocked)?|wd)?|d(?:ate|elim|irentriesattr|omainname|tablesize)|e(?:gid|nv|uid)|g(?:id|roup(?:list|s))|host(?:id|name)|iopolicy_np|l(?:ine|o(?:adavg|gin(?:_r)?))|mode|opt|p(?:a(?:gesize|ss)|eereid|g(?:id|rp)|id|pid|r(?:iority|ogname))|r(?:limit|usage)|s(?:groups_np|id|ubopt)?|u(?:id|sershell)|w(?:d|groups_np)?)|mtime(?:_r)?|rantpt)|h(?:eapsort(?:_b)?|ypot(?:f|l)?)|i(?:logb(?:f|l)?|n(?:dex|it(?:groups|state))|ruserok(?:_sa)?|s(?:a(?:l(?:num|pha)|scii|tty)|blank|cntrl|digit|graph|hexnumber|ideogram|lower|number|p(?:honogram|rint|unct)|rune|s(?:etugid|p(?:ace|ecial))|upper|xdigit))|j(?:0|1|n|rand48)|kill(?:pg)?|l(?:64a|abs|c(?:hown|ong48)|d(?:exp(?:f|l)?|iv)|gamma(?:f|l)?|ink|l(?:abs|div|r(?:int(?:f|l)?|ound(?:f|l)?))|o(?:c(?:al(?:econv|time(?:_r)?)|kf)|g(?:1(?:0(?:f|l)?|p(?:f|l)?)|2(?:f|l)?|b(?:f|l)?|f|l)?|ngjmp(?:error)?)|r(?:and48|int(?:f|l)?|ound(?:f|l)?)|seek)|m(?:a(?:jor|kedev|lloc)|b(?:len|stowcs|towc)|e(?:m(?:c(?:cpy|hr|mp|py)|m(?:em|ove)|set(?:_pattern(?:16|4|8))?)|rgesort(?:_b)?)|inor|k(?:dtemp|nod|stemp(?:_dprotected_np|s)?|t(?:emp|ime))|odf(?:f|l)?|rand48)|n(?:an(?:f|l|osleep)?|e(?:arbyint(?:f|l)?|xt(?:after(?:f|l)?|toward(?:f|l)?))|fssvc|ice|rand48)|open(?:_dprotected_np|x_np)?|p(?:a(?:thconf|use)|close|error|ipe|o(?:pen|six(?:2time|_(?:memalign|openpt))|w(?:f|l)?)|r(?:ead|intf|ofil)|s(?:elect|ignal|ort(?:_(?:b|r))?)|t(?:hread_(?:getugid_np|kill|s(?:etugid_np|igmask))|sname)|ut(?:c(?:_unlocked|har(?:_unlocked)?)?|env|s|w)|write)|qsort(?:_(?:b|r))?|r(?:a(?:dixsort|ise|nd(?:_r|om)?)|cmd(?:_af)?|e(?:a(?:d(?:link)?|l(?:loc(?:f)?|path))|boot|m(?:ainder(?:f|l)?|ove|quo(?:f|l)?)|name|voke|wind)|in(?:dex|t(?:f|l)?)|mdir|ound(?:f|l)?|resvport(?:_af)?|userok)|s(?:brk|ca(?:lb(?:ln(?:f|l)?|n(?:f|l)?)?|nf)|e(?:archfs|ed48|lect|t(?:attrlist|buf(?:fer)?|domainname|e(?:gid|nv|uid)|g(?:id|roups)|host(?:id|name)|iopolicy_np|jmp|key|l(?:inebuf|o(?:cale|gin))|mode|p(?:g(?:id|rp)|r(?:iority|ogname))|r(?:e(?:gid|uid)|gid|limit|uid)|s(?:groups_np|id|tate)|u(?:id|sershell)|vbuf|wgroups_np))|i(?:g(?:a(?:ction|ddset|ltstack)|block|delset|emptyset|fillset|hold|i(?:gnore|nterrupt|smember)|longjmp|nal|p(?:ause|ending|rocmask)|relse|s(?:et(?:jmp|mask)?|uspend)|vec|wait)|n(?:f|h(?:f|l)?|l)?)|leep|nprintf|printf|qrt(?:f|l)?|ra(?:dixsort|nd(?:48|dev|om(?:dev)?)?)|scanf|t(?:p(?:cpy|ncpy)|r(?:c(?:a(?:se(?:cmp|str)|t)|hr|mp|oll|py|spn)|dup|error(?:_r)?|ftime|l(?:c(?:at|py)|en)|mode|n(?:c(?:a(?:secmp|t)|mp|py)|dup|len|str)|p(?:brk|time)|rchr|s(?:ep|ignal|pn|tr)|to(?:d|f(?:flags)?|k(?:_r)?|l(?:d|l)?|q|u(?:l(?:l)?|q))|xfrm))|wa(?:b|pon)|y(?:mlink|nc|s(?:conf|tem)))|t(?:an(?:f|h(?:f|l)?|l)?|c(?:getpgrp|setpgrp)|empnam|gamma(?:f|l)?|ime(?:2posix|gm|local)?|mp(?:file|nam)|o(?:ascii|lower|upper)|runc(?:ate|f|l)?|ty(?:name(?:_r)?|slot)|zset(?:wall)?)|u(?:alarm|n(?:delete|getc|l(?:ink|ockpt)|setenv|whiteout)|sleep)|v(?:a(?:lloc|sprintf)|dprintf|f(?:ork|printf|scanf)|printf|s(?:canf|nprintf|printf|scanf))|w(?:ait(?:3|4|id|pid)?|c(?:stombs|tomb)|rite)|y(?:0|1|n)|zopen)\\b)"
+ "match": "(\\s*)(\\b(?:a(?:64l|b(?:ort|s)|c(?:c(?:ess(?:x_np)?|t)|os(?:f|h(?:f|l)?|l)?)|d(?:d_profil|jtime)|l(?:arm|loca)|rc4random(?:_(?:buf|stir|uniform))?|s(?:ctime(?:_r)?|in(?:f|h(?:f|l)?|l)?|printf)|t(?:an(?:2(?:f|l)?|f|h(?:f|l)?|l)?|exit(?:_b)?|o(?:f|i|l(?:l)?)))|b(?:c(?:mp|opy)|rk|s(?:d_signal|earch(?:_b)?)|zero)|c(?:brt(?:f|l)?|eil(?:f|l)?|get(?:c(?:ap|lose)|ent|first|match|n(?:ext|um)|s(?:et|tr)|ustr)|h(?:dir|own|root)|l(?:earerr|o(?:ck|se))|o(?:nfstr|pysign(?:f|l)?|s(?:f|h(?:f|l)?|l)?)|r(?:eat|ypt)|t(?:ermid_r|ime(?:_r)?))|d(?:evname(?:_r)?|i(?:fftime|spatch_(?:time|walltime)|v)|printf|rand48|up(?:2)?)|e(?:cvt|n(?:crypt|dusershell)|r(?:and48|f(?:c(?:f|l)?|f|l)?)|x(?:changedata|ec(?:l(?:e|p)?|v(?:P|e|p)?)|it|p(?:2(?:f|l)?|f|l|m1(?:f|l)?)?))|f(?:abs(?:f|l)?|c(?:h(?:dir|own)|lose|ntl|vt)|d(?:im(?:f|l)?|open)|e(?:of|rror)|f(?:l(?:agstostr|ush)|s(?:ctl|l)?)|get(?:attrlist|c|ln|pos|s)|ile(?:no|sec_(?:dup|free|get_property|init|query_property|set_property|unset_property))|l(?:o(?:ck(?:file)?|or(?:f|l)?)|s(?:l)?)|m(?:a(?:f|l|x(?:f|l)?)?|in(?:f|l)?|od(?:f|l)?|tcheck)|o(?:pen|rk)|p(?:athconf|rintf|u(?:rge|t(?:c|s)))|re(?:ad|open|xp(?:f|l)?)|s(?:c(?:anf|tl)|e(?:ek(?:o)?|t(?:attrlist|pos))|ync)|t(?:ell(?:o)?|r(?:uncate|ylockfile))|u(?:n(?:lockfile|open)|times)|write)|g(?:cvt|et(?:attrlist|bsize|c(?:_unlocked|har(?:_unlocked)?|wd)?|d(?:ate|elim|irentriesattr|omainname|tablesize)|e(?:gid|nv|uid)|g(?:id|roup(?:list|s))|host(?:id|name)|i(?:opolicy_np|timer)|l(?:ine|o(?:adavg|gin(?:_r)?))|mode|opt|p(?:a(?:gesize|ss)|eereid|g(?:id|rp)|id|pid|r(?:iority|ogname))|r(?:limit|usage)|s(?:groups_np|id|ubopt)?|timeofday|u(?:id|sershell)|w(?:d|groups_np)?)|mtime(?:_r)?|rantpt)|h(?:eapsort(?:_b)?|ypot(?:f|l)?)|i(?:logb(?:f|l)?|n(?:dex|it(?:groups|state))|ruserok(?:_sa)?|s(?:atty|setugid))|j(?:0|1|n|rand48)|kill(?:pg)?|l(?:64a|abs|c(?:hown|ong48)|d(?:exp(?:f|l)?|iv)|gamma(?:f|l)?|ink|l(?:abs|div|r(?:int(?:f|l)?|ound(?:f|l)?))|o(?:c(?:al(?:econv|time(?:_r)?)|kf)|g(?:1(?:0(?:f|l)?|p(?:f|l)?)|2(?:f|l)?|b(?:f|l)?|f|l)?|ngjmp(?:error)?)|r(?:and48|int(?:f|l)?|ound(?:f|l)?)|seek|utimes)|m(?:b(?:len|stowcs|towc)|e(?:m(?:c(?:cpy|hr|mp|py)|m(?:em|ove)|set(?:_pattern(?:16|4|8))?)|rgesort(?:_b)?)|k(?:dtemp|nod|stemp(?:_dprotected_np|s)?|t(?:emp|ime))|odf(?:f|l)?|rand48)|n(?:an(?:f|l|osleep)?|e(?:arbyint(?:f|l)?|xt(?:after(?:f|l)?|toward(?:f|l)?))|fssvc|ice|rand48)|open(?:_dprotected_np|x_np)?|p(?:a(?:thconf|use)|close|error|ipe|o(?:pen|six(?:2time|_openpt)|w(?:f|l)?)|r(?:ead|intf|ofil)|s(?:elect|ignal|ort(?:_(?:b|r))?)|t(?:hread_(?:getugid_np|kill|s(?:etugid_np|igmask))|sname)|ut(?:c(?:_unlocked|har(?:_unlocked)?)?|env|s|w)|write)|qsort(?:_(?:b|r))?|r(?:a(?:dixsort|ise|nd(?:_r|om)?)|cmd(?:_af)?|e(?:a(?:d(?:link)?|l(?:locf|path))|boot|m(?:ainder(?:f|l)?|ove|quo(?:f|l)?)|name|voke|wind)|in(?:dex|t(?:f|l)?)|mdir|ound(?:f|l)?|resvport(?:_af)?|userok)|s(?:brk|ca(?:lb(?:ln(?:f|l)?|n(?:f|l)?)?|nf)|e(?:archfs|ed48|lect|t(?:attrlist|buf(?:fer)?|domainname|e(?:gid|nv|uid)|g(?:id|roups)|host(?:id|name)|i(?:opolicy_np|timer)|jmp|key|l(?:inebuf|o(?:cale|gin))|mode|p(?:g(?:id|rp)|r(?:iority|ogname))|r(?:e(?:gid|uid)|gid|limit|uid)|s(?:groups_np|id|tate)|timeofday|u(?:id|sershell)|vbuf|wgroups_np))|i(?:g(?:a(?:ction|ddset|ltstack)|block|delset|emptyset|fillset|hold|i(?:gnore|nterrupt|smember)|longjmp|nal|p(?:ause|ending|rocmask)|relse|s(?:et(?:jmp|mask)?|uspend)|vec|wait)|md_muladd|n(?:f|h(?:f|l)?|l)?)|leep|nprintf|printf|qrt(?:f|l)?|ra(?:dixsort|nd(?:48|dev|om(?:dev)?)?)|scanf|t(?:p(?:cpy|ncpy)|r(?:c(?:a(?:se(?:cmp|str)|t)|hr|mp|oll|py|spn)|dup|error(?:_r)?|ftime|l(?:c(?:at|py)|en)|mode|n(?:c(?:a(?:secmp|t)|mp|py)|dup|len|str)|p(?:brk|time)|rchr|s(?:ep|ignal|pn|tr)|to(?:d|f(?:flags)?|k(?:_r)?|l(?:d|l)?|q|u(?:l(?:l)?|q))|xfrm))|wa(?:b|pon)|y(?:mlink|nc|s(?:conf|tem)))|t(?:an(?:f|h(?:f|l)?|l)?|c(?:getpgrp|setpgrp)|empnam|gamma(?:f|l)?|ime(?:2posix|gm|local)?|mp(?:file|nam)|runc(?:ate|f|l)?|ty(?:name(?:_r)?|slot)|zset(?:wall)?)|u(?:alarm|n(?:delete|getc|l(?:ink|ockpt)|setenv|whiteout)|sleep|times)|v(?:a(?:lloc|sprintf)|dprintf|f(?:ork|printf|scanf)|printf|s(?:canf|nprintf|printf|scanf))|w(?:ait(?:3|4|id|pid)?|c(?:stombs|tomb)|rite)|y(?:0|1|n)|zopen)\\b)"
},
{
"captures": {
@@ -461,10 +1005,10 @@
"name": "punctuation.whitespace.support.function.leading"
},
"2": {
- "name": "support.function.dispatch.c"
+ "name": "support.function.dispatch.10.14.c"
}
},
- "match": "(\\s*)(\\bdispatch_(?:a(?:fter(?:_f)?|pply(?:_f)?|sync(?:_f)?)|barrier_(?:async(?:_f)?|sync(?:_f)?)|cancel|data_(?:apply|c(?:opy_region|reate(?:_(?:concat|map|subrange))?)|get_size)|g(?:et_(?:context|global_queue|main_queue|specific)|roup_(?:async(?:_f)?|create|enter|leave|notify(?:_f)?|wait))|io_(?:barrier|c(?:lose|reate(?:_with_(?:io|path))?)|get_descriptor|read|set_(?:high_water|interval|low_water)|write)|main|notify|once(?:_f)?|queue_(?:create|get_(?:label|specific)|set_specific)|re(?:ad|lease|sume|tain)|s(?:e(?:maphore_(?:create|signal|wait)|t_(?:context|finalizer_f|target_queue))|ource_(?:c(?:ancel|reate)|get_(?:data|handle|mask)|merge_data|set_(?:cancel_handler(?:_f)?|event_handler(?:_f)?|registration_handler(?:_f)?|timer)|testcancel)|uspend|ync(?:_f)?)|testcancel|w(?:ait|rite))\\b)"
+ "match": "(\\s*)(\\bdispatch_(?:async_and_wait(?:_f)?|barrier_async_and_wait(?:_f)?|set_qos_class_floor|workloop_(?:create(?:_inactive)?|set_autorelease_frequency))\\b)"
},
{
"captures": {
@@ -472,10 +1016,10 @@
"name": "punctuation.whitespace.support.function.leading"
},
"2": {
- "name": "support.function.mac-classic.c"
+ "name": "support.function.dispatch.c"
}
},
- "match": "(\\s*)(\\bStrLength\\b)"
+ "match": "(\\s*)(\\bdispatch_(?:a(?:fter(?:_f)?|pply(?:_f)?|sync(?:_f)?)|barrier_(?:async(?:_f)?|sync(?:_f)?)|cancel|data_(?:apply|c(?:opy_region|reate(?:_(?:concat|map|subrange))?)|get_size)|g(?:et_(?:context|global_queue|main_queue|specific)|roup_(?:async(?:_f)?|create|enter|leave|notify(?:_f)?|wait))|io_(?:barrier|c(?:lose|reate(?:_with_(?:io|path))?)|get_descriptor|read|set_(?:high_water|interval|low_water)|write)|main|notify|once(?:_f)?|queue_(?:create|get_(?:label|specific)|set_specific)|re(?:ad|lease|sume|tain)|s(?:e(?:maphore_(?:create|signal|wait)|t_(?:context|finalizer_f|target_queue))|ource_(?:c(?:ancel|reate)|get_(?:data|handle|mask)|merge_data|set_(?:cancel_handler(?:_f)?|event_handler(?:_f)?|registration_handler(?:_f)?|timer)|testcancel)|uspend|ync(?:_f)?)|testcancel|w(?:ait|rite))\\b)"
},
{
"captures": {
@@ -494,10 +1038,21 @@
"name": "punctuation.whitespace.support.function.leading"
},
"2": {
- "name": "support.function.pthread.10.10.c"
+ "name": "support.function.quartz.10.11.c"
+ }
+ },
+ "match": "(\\s*)(\\bCG(?:ColorCreateCopyByMatchingToColorSpace|Event(?:PostToPid|TapCreateForPid)|ImageGetUTType)\\b)"
+ },
+ {
+ "captures": {
+ "1": {
+ "name": "punctuation.whitespace.support.function.leading"
+ },
+ "2": {
+ "name": "support.function.quartz.10.12.c"
}
},
- "match": "(\\s*)(\\bpthread_(?:attr_(?:get_qos_class_np|set_qos_class_np)|get_qos_class_np|override_qos_class_(?:end_np|start_np)|set_qos_class_self_np)\\b)"
+ "match": "(\\s*)(\\bCGColor(?:ConversionInfoCreate(?:FromList)?|Space(?:C(?:opy(?:ICCData|PropertyList)|reateWith(?:ICCData|PropertyList))|IsWideGamutRGB|SupportsOutput))\\b)"
},
{
"captures": {
@@ -505,10 +1060,10 @@
"name": "punctuation.whitespace.support.function.leading"
},
"2": {
- "name": "support.function.pthread.c"
+ "name": "support.function.quartz.10.13.c"
}
},
- "match": "(\\s*)(\\b(?:pthread_(?:at(?:fork|tr_(?:destroy|get(?:detachstate|guardsize|inheritsched|s(?:c(?:hedp(?:aram|olicy)|ope)|tack(?:addr|size)?))|init|set(?:detachstate|guardsize|inheritsched|s(?:c(?:hedp(?:aram|olicy)|ope)|tack(?:addr|size)?))))|c(?:ancel|ond(?:_(?:broadcast|destroy|init|signal(?:_thread_np)?|timedwait(?:_relative_np)?|wait)|attr_(?:destroy|getpshared|init|setpshared))|reate(?:_suspended_np)?)|detach|e(?:qual|xit)|from_mach_thread_np|get(?:_stack(?:addr_np|size_np)|concurrency|name_np|s(?:chedparam|pecific))|is_threaded_np|join|k(?:ey_(?:create|delete)|ill)|m(?:a(?:ch_thread_np|in_np)|utex(?:_(?:destroy|getprioceiling|init|lock|setprioceiling|trylock|unlock)|attr_(?:destroy|get(?:p(?:r(?:ioceiling|otocol)|shared)|type)|init|set(?:p(?:r(?:ioceiling|otocol)|shared)|type))))|once|rwlock(?:_(?:destroy|init|rdlock|try(?:rdlock|wrlock)|unlock|wrlock)|attr_(?:destroy|getpshared|init|setpshared))|s(?:e(?:lf|t(?:c(?:ancel(?:state|type)|oncurrency)|name_np|s(?:chedparam|pecific)))|igmask)|t(?:estcancel|hreadid_np)|yield_np)|sched_(?:get_priority_m(?:ax|in)|yield))\\b)"
+ "match": "(\\s*)(\\bCG(?:Color(?:ConversionInfoCreateFromListWithArguments|SpaceGetName)|DataProviderGetInfo|EventCreateScrollWheelEvent2|P(?:DF(?:ContextSetOutline|DocumentGet(?:AccessPermissions|Outline))|athApplyWithBlock))\\b)"
},
{
"captures": {
@@ -516,10 +1071,10 @@
"name": "punctuation.whitespace.support.function.leading"
},
"2": {
- "name": "support.function.quartz.10.11.c"
+ "name": "support.function.quartz.10.14.c"
}
},
- "match": "(\\s*)(\\bCG(?:ColorCreateCopyByMatchingToColorSpace|Event(?:PostToPid|TapCreateForPid)|ImageGetUTType)\\b)"
+ "match": "(\\s*)(\\bCG(?:ColorConversionInfoCreateWithOptions|ImageGet(?:ByteOrderInfo|PixelFormatInfo)|PDF(?:ArrayApplyBlock|DictionaryApplyBlock))\\b)"
},
{
"captures": {
@@ -527,10 +1082,10 @@
"name": "punctuation.whitespace.support.function.leading"
},
"2": {
- "name": "support.function.quartz.10.12.c"
+ "name": "support.function.quartz.10.15.c"
}
},
- "match": "(\\s*)(\\bCGColor(?:ConversionInfoCreate(?:FromList)?|Space(?:CopyICCData|IsWideGamutRGB|SupportsOutput))\\b)"
+ "match": "(\\s*)(\\bCG(?:ColorCreate(?:GenericGrayGamma2_2|SRGB)|PDF(?:Context(?:BeginTag|EndTag)|TagTypeGetName))\\b)"
},
{
"captures": {
@@ -563,7 +1118,7 @@
"name": "support.function.quartz.c"
}
},
- "match": "(\\s*)(\\bCG(?:A(?:cquireDisplayFadeReservation|ffineTransform(?:Concat|EqualToTransform|I(?:nvert|sIdentity)|Make(?:Rotation|Scale|Translation)?|Rotate|Scale|Translate)|ssociateMouseAndMouseCursorPosition)|B(?:eginDisplayConfiguration|itmapContext(?:Create(?:Image|WithData)?|Get(?:AlphaInfo|B(?:it(?:mapInfo|sPer(?:Component|Pixel))|ytesPerRow)|ColorSpace|Data|Height|Width)))|C(?:a(?:ncelDisplayConfiguration|ptureAllDisplays(?:WithOptions)?)|o(?:lor(?:C(?:onversionInfoGetTypeID|reate(?:Copy(?:WithAlpha)?|Generic(?:CMYK|Gray|RGB)|WithPattern)?)|EqualToColor|Get(?:Alpha|Co(?:lorSpace|mponents|nstantColor)|NumberOfComponents|Pattern|TypeID)|Re(?:lease|tain)|Space(?:C(?:opy(?:ICCProfile|Name)|reate(?:Calibrated(?:Gray|RGB)|Device(?:CMYK|Gray|RGB)|I(?:CCBased|ndexed)|Lab|Pattern|With(?:ICCProfile|Name|PlatformColorSpace)))|Get(?:BaseColorSpace|ColorTable(?:Count)?|Model|NumberOfComponents|TypeID)|Re(?:lease|tain)))|mpleteDisplayConfiguration|n(?:figureDisplay(?:FadeEffect|MirrorOfDisplay|Origin|StereoOperation|WithDisplayMode)|text(?:Add(?:Arc(?:ToPoint)?|CurveToPoint|EllipseInRect|Line(?:ToPoint|s)|Path|QuadCurveToPoint|Rect(?:s)?)|Begin(?:Pa(?:ge|th)|TransparencyLayer(?:WithRect)?)|C(?:l(?:earRect|ip(?:To(?:Mask|Rect(?:s)?))?|osePath)|o(?:n(?:catCTM|vert(?:PointTo(?:DeviceSpace|UserSpace)|RectTo(?:DeviceSpace|UserSpace)|SizeTo(?:DeviceSpace|UserSpace)))|pyPath))|Draw(?:Image|L(?:ayer(?:AtPoint|InRect)|inearGradient)|P(?:DFPage|ath)|RadialGradient|Shading|TiledImage)|E(?:O(?:Clip|FillPath)|nd(?:Page|TransparencyLayer))|F(?:ill(?:EllipseInRect|Path|Rect(?:s)?)|lush)|Get(?:C(?:TM|lipBoundingBox)|InterpolationQuality|Path(?:BoundingBox|CurrentPoint)|T(?:ext(?:Matrix|Position)|ypeID)|UserSpaceToDeviceSpaceTransform)|IsPathEmpty|MoveToPoint|PathContainsPoint|R(?:e(?:lease|placePathWithStrokedPath|storeGState|tain)|otateCTM)|S(?:aveGState|caleCTM|et(?:Al(?:lows(?:Antialiasing|FontS(?:moothing|ubpixel(?:Positioning|Quantization)))|pha)|BlendMode|C(?:MYK(?:FillColor|StrokeColor)|haracterSpacing)|F(?:ill(?:Color(?:Space|WithColor)?|Pattern)|latness|ont(?:Size)?)|Gray(?:FillColor|StrokeColor)|InterpolationQuality|Line(?:Cap|Dash|Join|Width)|MiterLimit|PatternPhase|R(?:GB(?:FillColor|StrokeColor)|enderingIntent)|S(?:h(?:adow(?:WithColor)?|ould(?:Antialias|S(?:moothFonts|ubpixel(?:PositionFonts|QuantizeFonts))))|troke(?:Color(?:Space|WithColor)?|Pattern))|Text(?:DrawingMode|Matrix|Position))|howGlyphsAtPositions|troke(?:EllipseInRect|LineSegments|Path|Rect(?:WithWidth)?)|ynchronize)|TranslateCTM))))|D(?:ata(?:Consumer(?:Create(?:With(?:CFData|URL))?|GetTypeID|Re(?:lease|tain))|Provider(?:C(?:opyData|reate(?:Direct|Sequential|With(?:CFData|Data|Filename|URL)))|GetTypeID|Re(?:lease|tain)))|isplay(?:Bounds|C(?:apture(?:WithOptions)?|opy(?:AllDisplayModes|ColorSpace|DisplayMode)|reateImage(?:ForRect)?)|Fade|G(?:ammaTableCapacity|etDrawingContext)|HideCursor|I(?:DToOpenGLDisplayMask|s(?:A(?:ctive|lwaysInMirrorSet|sleep)|Builtin|In(?:HWMirrorSet|MirrorSet)|Main|Online|Stereo))|M(?:irrorsDisplay|o(?:de(?:Get(?:Height|IO(?:DisplayModeID|Flags)|RefreshRate|TypeID|Width)|IsUsableForDesktopGUI|Re(?:lease|tain)|lNumber)|veCursorToPoint))|P(?:ixels(?:High|Wide)|rimaryDisplay)|R(?:e(?:gisterReconfigurationCallback|lease|moveReconfigurationCallback|storeColorSyncSettings)|otation)|S(?:creenSize|e(?:rialNumber|t(?:DisplayMode|StereoOperation))|howCursor)|U(?:nitNumber|sesOpenGLAcceleration)|VendorNumber))|Event(?:Create(?:Copy|Data|FromData|KeyboardEvent|MouseEvent|S(?:crollWheelEvent|ourceFromEvent))?|Get(?:DoubleValueField|Flags|IntegerValueField|Location|T(?:imestamp|ype(?:ID)?)|UnflippedLocation)|Keyboard(?:GetUnicodeString|SetUnicodeString)|Post(?:ToPSN)?|S(?:et(?:DoubleValueField|Flags|IntegerValueField|Location|Source|T(?:imestamp|ype))|ource(?:ButtonState|C(?:ounterForEventType|reate)|FlagsState|Get(?:KeyboardType|LocalEvents(?:FilterDuringSuppressionState|SuppressionInterval)|PixelsPerLine|SourceStateID|TypeID|UserData)|KeyState|Se(?:condsSinceLastEventType|t(?:KeyboardType|LocalEvents(?:FilterDuringSuppressionState|SuppressionInterval)|PixelsPerLine|UserData))))|Tap(?:Create(?:ForPSN)?|Enable|IsEnabled|PostEvent))|F(?:ont(?:C(?:anCreatePostScriptSubset|opy(?:FullName|GlyphNameForGlyph|PostScriptName|Table(?:ForTag|Tags)|Variation(?:Axes|s))|reate(?:CopyWithVariations|PostScript(?:Encoding|Subset)|With(?:DataProvider|FontName)))|Get(?:Ascent|CapHeight|Descent|FontBBox|Glyph(?:Advances|BBoxes|WithGlyphName)|ItalicAngle|Leading|NumberOfGlyphs|StemV|TypeID|UnitsPerEm|XHeight)|Re(?:lease|tain))|unction(?:Create|GetTypeID|Re(?:lease|tain)))|G(?:et(?:ActiveDisplayList|Display(?:TransferBy(?:Formula|Table)|sWith(?:OpenGLDisplayMask|Point|Rect))|EventTapList|LastMouseDelta|OnlineDisplayList)|radient(?:CreateWithColor(?:Components|s)|GetTypeID|Re(?:lease|tain)))|Image(?:Create(?:Copy(?:WithColorSpace)?|With(?:ImageInRect|JPEGDataProvider|Mask(?:ingColors)?|PNGDataProvider))?|Get(?:AlphaInfo|B(?:it(?:mapInfo|sPer(?:Component|Pixel))|ytesPerRow)|ColorSpace|D(?:ataProvider|ecode)|Height|RenderingIntent|ShouldInterpolate|TypeID|Width)|IsMask|MaskCreate|Re(?:lease|tain))|Layer(?:CreateWithContext|Get(?:Context|Size|TypeID)|Re(?:lease|tain))|MainDisplayID|OpenGLDisplayMaskToDisplayID|P(?:DF(?:ArrayGet(?:Array|Boolean|Count|Dictionary|Integer|N(?:ame|u(?:ll|mber))|Object|Str(?:eam|ing))|Conte(?:ntStream(?:CreateWith(?:Page|Stream)|Get(?:Resource|Streams)|Re(?:lease|tain))|xt(?:AddD(?:estinationAtPoint|ocumentMetadata)|BeginPage|C(?:lose|reate(?:WithURL)?)|EndPage|Set(?:DestinationForRect|URLForRect)))|D(?:ictionary(?:ApplyFunction|Get(?:Array|Boolean|Count|Dictionary|Integer|N(?:ame|umber)|Object|Str(?:eam|ing)))|ocument(?:Allows(?:Copying|Printing)|CreateWith(?:Provider|URL)|Get(?:Catalog|I(?:D|nfo)|NumberOfPages|Page|TypeID|Version)|Is(?:Encrypted|Unlocked)|Re(?:lease|tain)|UnlockWithPassword))|O(?:bjectGet(?:Type|Value)|peratorTable(?:Create|Re(?:lease|tain)|SetCallback))|Page(?:Get(?:BoxRect|D(?:ictionary|ocument|rawingTransform)|PageNumber|RotationAngle|TypeID)|Re(?:lease|tain))|S(?:canner(?:Create|GetContentStream|Pop(?:Array|Boolean|Dictionary|Integer|N(?:ame|umber)|Object|Str(?:eam|ing))|Re(?:lease|tain)|Scan)|tr(?:eam(?:CopyData|GetDictionary)|ing(?:Copy(?:Date|TextString)|Get(?:BytePtr|Length)))))|SConverter(?:Abort|C(?:onvert|reate)|GetTypeID|IsConverting)|at(?:h(?:A(?:dd(?:Arc(?:ToPoint)?|CurveToPoint|EllipseInRect|Line(?:ToPoint|s)|Path|QuadCurveToPoint|Re(?:ct(?:s)?|lativeArc))|pply)|C(?:loseSubpath|ontainsPoint|reate(?:Copy(?:By(?:DashingPath|StrokingPath|TransformingPath))?|Mutable(?:Copy(?:ByTransformingPath)?)?|With(?:EllipseInRect|Rect)))|EqualToPath|Get(?:BoundingBox|CurrentPoint|PathBoundingBox|TypeID)|Is(?:Empty|Rect)|MoveToPoint|Re(?:lease|tain))|tern(?:Create|GetTypeID|Re(?:lease|tain)))|oint(?:ApplyAffineTransform|CreateDictionaryRepresentation|EqualToPoint|Make(?:WithDictionaryRepresentation)?))|Re(?:ct(?:ApplyAffineTransform|C(?:ontains(?:Point|Rect)|reateDictionaryRepresentation)|Divide|EqualToRect|Get(?:Height|M(?:ax(?:X|Y)|i(?:d(?:X|Y)|n(?:X|Y)))|Width)|I(?:n(?:set|te(?:gral|rsect(?:ion|sRect)))|s(?:Empty|Infinite|Null))|Make(?:WithDictionaryRepresentation)?|Offset|Standardize|Union)|lease(?:AllDisplays|DisplayFadeReservation)|storePermanentDisplayConfiguration)|S(?:e(?:ssionCopyCurrentDictionary|tDisplayTransferBy(?:ByteTable|Formula|Table))|h(?:ading(?:Create(?:Axial|Radial)|GetTypeID|Re(?:lease|tain))|ieldingWindow(?:ID|Level))|ize(?:ApplyAffineTransform|CreateDictionaryRepresentation|EqualToSize|Make(?:WithDictionaryRepresentation)?))|VectorMake|W(?:arpMouseCursorPosition|indowL(?:evelForKey|istC(?:opyWindowInfo|reate(?:DescriptionFromArray|Image(?:FromArray)?)?))))\\b)"
+ "match": "(\\s*)(\\bCG(?:A(?:cquireDisplayFadeReservation|ffineTransform(?:Concat|EqualToTransform|I(?:nvert|sIdentity)|Make(?:Rotation|Scale|Translation)?|Rotate|Scale|Translate)|ssociateMouseAndMouseCursorPosition)|B(?:eginDisplayConfiguration|itmapContext(?:Create(?:Image|WithData)?|Get(?:AlphaInfo|B(?:it(?:mapInfo|sPer(?:Component|Pixel))|ytesPerRow)|ColorSpace|Data|Height|Width)))|C(?:a(?:ncelDisplayConfiguration|ptureAllDisplays(?:WithOptions)?)|o(?:lor(?:C(?:onversionInfoGetTypeID|reate(?:Copy(?:WithAlpha)?|Generic(?:CMYK|Gray|RGB)|WithPattern)?)|EqualToColor|Get(?:Alpha|Co(?:lorSpace|mponents|nstantColor)|NumberOfComponents|Pattern|TypeID)|Re(?:lease|tain)|Space(?:C(?:opyName|reate(?:Calibrated(?:Gray|RGB)|Device(?:CMYK|Gray|RGB)|I(?:CCBased|ndexed)|Lab|Pattern|With(?:Name|PlatformColorSpace)))|Get(?:BaseColorSpace|ColorTable(?:Count)?|Model|NumberOfComponents|TypeID)|Re(?:lease|tain)))|mpleteDisplayConfiguration|n(?:figureDisplay(?:FadeEffect|MirrorOfDisplay|Origin|StereoOperation|WithDisplayMode)|text(?:Add(?:Arc(?:ToPoint)?|CurveToPoint|EllipseInRect|Line(?:ToPoint|s)|Path|QuadCurveToPoint|Rect(?:s)?)|Begin(?:Pa(?:ge|th)|TransparencyLayer(?:WithRect)?)|C(?:l(?:earRect|ip(?:To(?:Mask|Rect(?:s)?))?|osePath)|o(?:n(?:catCTM|vert(?:PointTo(?:DeviceSpace|UserSpace)|RectTo(?:DeviceSpace|UserSpace)|SizeTo(?:DeviceSpace|UserSpace)))|pyPath))|Draw(?:Image|L(?:ayer(?:AtPoint|InRect)|inearGradient)|P(?:DFPage|ath)|RadialGradient|Shading|TiledImage)|E(?:O(?:Clip|FillPath)|nd(?:Page|TransparencyLayer))|F(?:ill(?:EllipseInRect|Path|Rect(?:s)?)|lush)|Get(?:C(?:TM|lipBoundingBox)|InterpolationQuality|Path(?:BoundingBox|CurrentPoint)|T(?:ext(?:Matrix|Position)|ypeID)|UserSpaceToDeviceSpaceTransform)|IsPathEmpty|MoveToPoint|PathContainsPoint|R(?:e(?:lease|placePathWithStrokedPath|s(?:etClip|toreGState)|tain)|otateCTM)|S(?:aveGState|caleCTM|et(?:Al(?:lows(?:Antialiasing|FontS(?:moothing|ubpixel(?:Positioning|Quantization)))|pha)|BlendMode|C(?:MYK(?:FillColor|StrokeColor)|haracterSpacing)|F(?:ill(?:Color(?:Space|WithColor)?|Pattern)|latness|ont(?:Size)?)|Gray(?:FillColor|StrokeColor)|InterpolationQuality|Line(?:Cap|Dash|Join|Width)|MiterLimit|PatternPhase|R(?:GB(?:FillColor|StrokeColor)|enderingIntent)|S(?:h(?:adow(?:WithColor)?|ould(?:Antialias|S(?:moothFonts|ubpixel(?:PositionFonts|QuantizeFonts))))|troke(?:Color(?:Space|WithColor)?|Pattern))|Text(?:DrawingMode|Matrix|Position))|howGlyphsAtPositions|troke(?:EllipseInRect|LineSegments|Path|Rect(?:WithWidth)?)|ynchronize)|TranslateCTM))))|D(?:ata(?:Consumer(?:Create(?:With(?:CFData|URL))?|GetTypeID|Re(?:lease|tain))|Provider(?:C(?:opyData|reate(?:Direct|Sequential|With(?:CFData|Data|Filename|URL)))|GetTypeID|Re(?:lease|tain)))|isplay(?:Bounds|C(?:apture(?:WithOptions)?|opy(?:AllDisplayModes|ColorSpace|DisplayMode)|reateImage(?:ForRect)?)|Fade|G(?:ammaTableCapacity|etDrawingContext)|HideCursor|I(?:DToOpenGLDisplayMask|s(?:A(?:ctive|lwaysInMirrorSet|sleep)|Builtin|In(?:HWMirrorSet|MirrorSet)|Main|Online|Stereo))|M(?:irrorsDisplay|o(?:de(?:Get(?:Height|IO(?:DisplayModeID|Flags)|RefreshRate|TypeID|Width)|IsUsableForDesktopGUI|Re(?:lease|tain)|lNumber)|veCursorToPoint))|P(?:ixels(?:High|Wide)|rimaryDisplay)|R(?:e(?:gisterReconfigurationCallback|lease|moveReconfigurationCallback|storeColorSyncSettings)|otation)|S(?:creenSize|e(?:rialNumber|t(?:DisplayMode|StereoOperation))|howCursor)|U(?:nitNumber|sesOpenGLAcceleration)|VendorNumber))|Event(?:Create(?:Copy|Data|FromData|KeyboardEvent|MouseEvent|S(?:crollWheelEvent|ourceFromEvent))?|Get(?:DoubleValueField|Flags|IntegerValueField|Location|T(?:imestamp|ype(?:ID)?)|UnflippedLocation)|Keyboard(?:GetUnicodeString|SetUnicodeString)|Post(?:ToPSN)?|S(?:et(?:DoubleValueField|Flags|IntegerValueField|Location|Source|T(?:imestamp|ype))|ource(?:ButtonState|C(?:ounterForEventType|reate)|FlagsState|Get(?:KeyboardType|LocalEvents(?:FilterDuringSuppressionState|SuppressionInterval)|PixelsPerLine|SourceStateID|TypeID|UserData)|KeyState|Se(?:condsSinceLastEventType|t(?:KeyboardType|LocalEvents(?:FilterDuringSuppressionState|SuppressionInterval)|PixelsPerLine|UserData))))|Tap(?:Create(?:ForPSN)?|Enable|IsEnabled|PostEvent))|F(?:ont(?:C(?:anCreatePostScriptSubset|opy(?:FullName|GlyphNameForGlyph|PostScriptName|Table(?:ForTag|Tags)|Variation(?:Axes|s))|reate(?:CopyWithVariations|PostScript(?:Encoding|Subset)|With(?:DataProvider|FontName)))|Get(?:Ascent|CapHeight|Descent|FontBBox|Glyph(?:Advances|BBoxes|WithGlyphName)|ItalicAngle|Leading|NumberOfGlyphs|StemV|TypeID|UnitsPerEm|XHeight)|Re(?:lease|tain))|unction(?:Create|GetTypeID|Re(?:lease|tain)))|G(?:et(?:ActiveDisplayList|Display(?:TransferBy(?:Formula|Table)|sWith(?:OpenGLDisplayMask|Point|Rect))|EventTapList|LastMouseDelta|OnlineDisplayList)|radient(?:CreateWithColor(?:Components|s)|GetTypeID|Re(?:lease|tain)))|Image(?:Create(?:Copy(?:WithColorSpace)?|With(?:ImageInRect|JPEGDataProvider|Mask(?:ingColors)?|PNGDataProvider))?|Get(?:AlphaInfo|B(?:it(?:mapInfo|sPer(?:Component|Pixel))|ytesPerRow)|ColorSpace|D(?:ataProvider|ecode)|Height|RenderingIntent|ShouldInterpolate|TypeID|Width)|IsMask|MaskCreate|Re(?:lease|tain))|Layer(?:CreateWithContext|Get(?:Context|Size|TypeID)|Re(?:lease|tain))|MainDisplayID|OpenGLDisplayMaskToDisplayID|P(?:DF(?:ArrayGet(?:Array|Boolean|Count|Dictionary|Integer|N(?:ame|u(?:ll|mber))|Object|Str(?:eam|ing))|Conte(?:ntStream(?:CreateWith(?:Page|Stream)|Get(?:Resource|Streams)|Re(?:lease|tain))|xt(?:AddD(?:estinationAtPoint|ocumentMetadata)|BeginPage|C(?:lose|reate(?:WithURL)?)|EndPage|Set(?:DestinationForRect|URLForRect)))|D(?:ictionary(?:ApplyFunction|Get(?:Array|Boolean|Count|Dictionary|Integer|N(?:ame|umber)|Object|Str(?:eam|ing)))|ocument(?:Allows(?:Copying|Printing)|CreateWith(?:Provider|URL)|Get(?:Catalog|I(?:D|nfo)|NumberOfPages|Page|TypeID|Version)|Is(?:Encrypted|Unlocked)|Re(?:lease|tain)|UnlockWithPassword))|O(?:bjectGet(?:Type|Value)|peratorTable(?:Create|Re(?:lease|tain)|SetCallback))|Page(?:Get(?:BoxRect|D(?:ictionary|ocument|rawingTransform)|PageNumber|RotationAngle|TypeID)|Re(?:lease|tain))|S(?:canner(?:Create|GetContentStream|Pop(?:Array|Boolean|Dictionary|Integer|N(?:ame|umber)|Object|Str(?:eam|ing))|Re(?:lease|tain)|Scan)|tr(?:eam(?:CopyData|GetDictionary)|ing(?:Copy(?:Date|TextString)|Get(?:BytePtr|Length)))))|SConverter(?:Abort|C(?:onvert|reate)|GetTypeID|IsConverting)|at(?:h(?:A(?:dd(?:Arc(?:ToPoint)?|CurveToPoint|EllipseInRect|Line(?:ToPoint|s)|Path|QuadCurveToPoint|Re(?:ct(?:s)?|lativeArc))|pply)|C(?:loseSubpath|ontainsPoint|reate(?:Copy(?:By(?:DashingPath|StrokingPath|TransformingPath))?|Mutable(?:Copy(?:ByTransformingPath)?)?|With(?:EllipseInRect|Rect)))|EqualToPath|Get(?:BoundingBox|CurrentPoint|PathBoundingBox|TypeID)|Is(?:Empty|Rect)|MoveToPoint|Re(?:lease|tain))|tern(?:Create|GetTypeID|Re(?:lease|tain)))|oint(?:ApplyAffineTransform|CreateDictionaryRepresentation|EqualToPoint|Make(?:WithDictionaryRepresentation)?))|Re(?:ct(?:ApplyAffineTransform|C(?:ontains(?:Point|Rect)|reateDictionaryRepresentation)|Divide|EqualToRect|Get(?:Height|M(?:ax(?:X|Y)|i(?:d(?:X|Y)|n(?:X|Y)))|Width)|I(?:n(?:set|te(?:gral|rsect(?:ion|sRect)))|s(?:Empty|Infinite|Null))|Make(?:WithDictionaryRepresentation)?|Offset|Standardize|Union)|lease(?:AllDisplays|DisplayFadeReservation)|storePermanentDisplayConfiguration)|S(?:e(?:ssionCopyCurrentDictionary|tDisplayTransferBy(?:ByteTable|Formula|Table))|h(?:ading(?:Create(?:Axial|Radial)|GetTypeID|Re(?:lease|tain))|ieldingWindow(?:ID|Level))|ize(?:ApplyAffineTransform|CreateDictionaryRepresentation|EqualToSize|Make(?:WithDictionaryRepresentation)?))|VectorMake|W(?:arpMouseCursorPosition|indowL(?:evelForKey|istC(?:opyWindowInfo|reate(?:DescriptionFromArray|Image(?:FromArray)?)?))))\\b)"
}
]
}
diff --git a/extensions/cpp/test/colorize-fixtures/test-92369.cpp b/extensions/cpp/test/colorize-fixtures/test-92369.cpp
new file mode 100644
index 0000000000000..b91dec67af647
--- /dev/null
+++ b/extensions/cpp/test/colorize-fixtures/test-92369.cpp
@@ -0,0 +1,3 @@
+std::tuple_element<0, std::pair, pugi::xml_node, std::less >, std::allocator, pugi::xml_node> > > > >::type dnode
+
+std::_Rb_tree_iterator, pugi::xml_node, std::less >, std::allocator, pugi::xml_node> > > > > > dnode_it = dnodes_.find(uid.position)
diff --git a/extensions/cpp/test/colorize-fixtures/test.cpp b/extensions/cpp/test/colorize-fixtures/test.cpp
index c3c3c10a953c2..13ec47a554cc4 100644
--- a/extensions/cpp/test/colorize-fixtures/test.cpp
+++ b/extensions/cpp/test/colorize-fixtures/test.cpp
@@ -16,7 +16,13 @@ void Rectangle::set_values (int x, int y) {
height = y;
}
+long double operator "" _w(long double);
+#define MY_MACRO(a, b)
+
int main () {
+ 1.2_w; // calls operator "" _w(1.2L)
+ asm("movl %a %b");
+ MY_MACRO(1, 2);
Rectangle rect;
rect.set_values (3,4);
cout << "area: " << rect.area();
diff --git a/extensions/cpp/test/colorize-results/test-23630_cpp.json b/extensions/cpp/test/colorize-results/test-23630_cpp.json
index 08d81e6afff9b..4ffc7f01f9878 100644
--- a/extensions/cpp/test/colorize-results/test-23630_cpp.json
+++ b/extensions/cpp/test/colorize-results/test-23630_cpp.json
@@ -36,10 +36,10 @@
"c": "_UCRT",
"t": "source.cpp meta.preprocessor.conditional.cpp entity.name.function.preprocessor.cpp",
"r": {
- "dark_plus": "entity.name.function: #DCDCAA",
- "light_plus": "entity.name.function: #795E26",
- "dark_vs": "meta.preprocessor: #569CD6",
- "light_vs": "meta.preprocessor: #0000FF",
+ "dark_plus": "entity.name.function.preprocessor: #569CD6",
+ "light_plus": "entity.name.function.preprocessor: #0000FF",
+ "dark_vs": "entity.name.function.preprocessor: #569CD6",
+ "light_vs": "entity.name.function.preprocessor: #0000FF",
"hc_black": "entity.name.function: #DCDCAA"
}
},
@@ -91,10 +91,10 @@
"c": "_UCRT",
"t": "source.cpp meta.preprocessor.macro.cpp entity.name.function.preprocessor.cpp",
"r": {
- "dark_plus": "entity.name.function: #DCDCAA",
- "light_plus": "entity.name.function: #795E26",
- "dark_vs": "meta.preprocessor: #569CD6",
- "light_vs": "meta.preprocessor: #0000FF",
+ "dark_plus": "entity.name.function.preprocessor: #569CD6",
+ "light_plus": "entity.name.function.preprocessor: #0000FF",
+ "dark_vs": "entity.name.function.preprocessor: #569CD6",
+ "light_vs": "entity.name.function.preprocessor: #0000FF",
"hc_black": "entity.name.function: #DCDCAA"
}
},
diff --git a/extensions/cpp/test/colorize-results/test-23850_cpp.json b/extensions/cpp/test/colorize-results/test-23850_cpp.json
index 4ba6b59dc9bc6..a97f9612665a8 100644
--- a/extensions/cpp/test/colorize-results/test-23850_cpp.json
+++ b/extensions/cpp/test/colorize-results/test-23850_cpp.json
@@ -36,10 +36,10 @@
"c": "_UCRT",
"t": "source.cpp meta.preprocessor.conditional.cpp entity.name.function.preprocessor.cpp",
"r": {
- "dark_plus": "entity.name.function: #DCDCAA",
- "light_plus": "entity.name.function: #795E26",
- "dark_vs": "meta.preprocessor: #569CD6",
- "light_vs": "meta.preprocessor: #0000FF",
+ "dark_plus": "entity.name.function.preprocessor: #569CD6",
+ "light_plus": "entity.name.function.preprocessor: #0000FF",
+ "dark_vs": "entity.name.function.preprocessor: #569CD6",
+ "light_vs": "entity.name.function.preprocessor: #0000FF",
"hc_black": "entity.name.function: #DCDCAA"
}
},
@@ -80,10 +80,10 @@
"c": "_UCRT",
"t": "source.cpp meta.preprocessor.macro.cpp entity.name.function.preprocessor.cpp",
"r": {
- "dark_plus": "entity.name.function: #DCDCAA",
- "light_plus": "entity.name.function: #795E26",
- "dark_vs": "meta.preprocessor: #569CD6",
- "light_vs": "meta.preprocessor: #0000FF",
+ "dark_plus": "entity.name.function.preprocessor: #569CD6",
+ "light_plus": "entity.name.function.preprocessor: #0000FF",
+ "dark_vs": "entity.name.function.preprocessor: #569CD6",
+ "light_vs": "entity.name.function.preprocessor: #0000FF",
"hc_black": "entity.name.function: #DCDCAA"
}
},
diff --git a/extensions/cpp/test/colorize-results/test-78769_cpp.json b/extensions/cpp/test/colorize-results/test-78769_cpp.json
index 1d82d5c4e43e4..8284688060362 100644
--- a/extensions/cpp/test/colorize-results/test-78769_cpp.json
+++ b/extensions/cpp/test/colorize-results/test-78769_cpp.json
@@ -36,10 +36,10 @@
"c": "DOCTEST_IMPLEMENT_FIXTURE",
"t": "source.cpp meta.preprocessor.macro.cpp entity.name.function.preprocessor.cpp",
"r": {
- "dark_plus": "entity.name.function: #DCDCAA",
- "light_plus": "entity.name.function: #795E26",
- "dark_vs": "meta.preprocessor: #569CD6",
- "light_vs": "meta.preprocessor: #0000FF",
+ "dark_plus": "entity.name.function.preprocessor: #569CD6",
+ "light_plus": "entity.name.function.preprocessor: #0000FF",
+ "dark_vs": "entity.name.function.preprocessor: #569CD6",
+ "light_vs": "entity.name.function.preprocessor: #0000FF",
"hc_black": "entity.name.function: #DCDCAA"
}
},
@@ -191,7 +191,7 @@
"t": "source.cpp meta.preprocessor.macro.cpp constant.character.escape.line-continuation.cpp",
"r": {
"dark_plus": "constant.character.escape: #D7BA7D",
- "light_plus": "constant.character.escape: #FF0000",
+ "light_plus": "constant.character.escape: #EE0000",
"dark_vs": "meta.preprocessor: #569CD6",
"light_vs": "meta.preprocessor: #0000FF",
"hc_black": "constant.character: #569CD6"
@@ -257,7 +257,7 @@
"t": "source.cpp meta.preprocessor.macro.cpp meta.block.namespace.cpp meta.body.namespace.cpp constant.character.escape.line-continuation.cpp",
"r": {
"dark_plus": "constant.character.escape: #D7BA7D",
- "light_plus": "constant.character.escape: #FF0000",
+ "light_plus": "constant.character.escape: #EE0000",
"dark_vs": "meta.preprocessor: #569CD6",
"light_vs": "meta.preprocessor: #0000FF",
"hc_black": "constant.character: #569CD6"
@@ -389,7 +389,7 @@
"t": "source.cpp meta.preprocessor.macro.cpp meta.block.namespace.cpp meta.body.namespace.cpp meta.block.struct.cpp constant.character.escape.line-continuation.cpp",
"r": {
"dark_plus": "constant.character.escape: #D7BA7D",
- "light_plus": "constant.character.escape: #FF0000",
+ "light_plus": "constant.character.escape: #EE0000",
"dark_vs": "meta.preprocessor: #569CD6",
"light_vs": "meta.preprocessor: #0000FF",
"hc_black": "constant.character: #569CD6"
@@ -433,7 +433,7 @@
"t": "source.cpp meta.preprocessor.macro.cpp meta.block.namespace.cpp meta.body.namespace.cpp meta.block.struct.cpp meta.body.struct.cpp constant.character.escape.line-continuation.cpp",
"r": {
"dark_plus": "constant.character.escape: #D7BA7D",
- "light_plus": "constant.character.escape: #FF0000",
+ "light_plus": "constant.character.escape: #EE0000",
"dark_vs": "meta.preprocessor: #569CD6",
"light_vs": "meta.preprocessor: #0000FF",
"hc_black": "constant.character: #569CD6"
@@ -532,7 +532,7 @@
"t": "source.cpp meta.preprocessor.macro.cpp meta.block.namespace.cpp meta.body.namespace.cpp meta.block.struct.cpp meta.body.struct.cpp constant.character.escape.line-continuation.cpp",
"r": {
"dark_plus": "constant.character.escape: #D7BA7D",
- "light_plus": "constant.character.escape: #FF0000",
+ "light_plus": "constant.character.escape: #EE0000",
"dark_vs": "meta.preprocessor: #569CD6",
"light_vs": "meta.preprocessor: #0000FF",
"hc_black": "constant.character: #569CD6"
@@ -587,7 +587,7 @@
"t": "source.cpp meta.preprocessor.macro.cpp meta.block.namespace.cpp meta.body.namespace.cpp constant.character.escape.line-continuation.cpp",
"r": {
"dark_plus": "constant.character.escape: #D7BA7D",
- "light_plus": "constant.character.escape: #FF0000",
+ "light_plus": "constant.character.escape: #EE0000",
"dark_vs": "meta.preprocessor: #569CD6",
"light_vs": "meta.preprocessor: #0000FF",
"hc_black": "constant.character: #569CD6"
@@ -719,7 +719,7 @@
"t": "source.cpp meta.preprocessor.macro.cpp meta.block.namespace.cpp meta.body.namespace.cpp meta.function.definition.cpp meta.body.function.definition.cpp constant.character.escape.line-continuation.cpp",
"r": {
"dark_plus": "constant.character.escape: #D7BA7D",
- "light_plus": "constant.character.escape: #FF0000",
+ "light_plus": "constant.character.escape: #EE0000",
"dark_vs": "meta.preprocessor: #569CD6",
"light_vs": "meta.preprocessor: #0000FF",
"hc_black": "constant.character: #569CD6"
@@ -763,7 +763,7 @@
"t": "source.cpp meta.preprocessor.macro.cpp meta.block.namespace.cpp meta.body.namespace.cpp meta.function.definition.cpp meta.body.function.definition.cpp constant.character.escape.line-continuation.cpp",
"r": {
"dark_plus": "constant.character.escape: #D7BA7D",
- "light_plus": "constant.character.escape: #FF0000",
+ "light_plus": "constant.character.escape: #EE0000",
"dark_vs": "meta.preprocessor: #569CD6",
"light_vs": "meta.preprocessor: #0000FF",
"hc_black": "constant.character: #569CD6"
@@ -862,7 +862,7 @@
"t": "source.cpp meta.preprocessor.macro.cpp meta.block.namespace.cpp meta.body.namespace.cpp meta.function.definition.cpp meta.body.function.definition.cpp constant.character.escape.line-continuation.cpp",
"r": {
"dark_plus": "constant.character.escape: #D7BA7D",
- "light_plus": "constant.character.escape: #FF0000",
+ "light_plus": "constant.character.escape: #EE0000",
"dark_vs": "meta.preprocessor: #569CD6",
"light_vs": "meta.preprocessor: #0000FF",
"hc_black": "constant.character: #569CD6"
@@ -906,7 +906,7 @@
"t": "source.cpp meta.preprocessor.macro.cpp meta.block.namespace.cpp meta.body.namespace.cpp constant.character.escape.line-continuation.cpp",
"r": {
"dark_plus": "constant.character.escape: #D7BA7D",
- "light_plus": "constant.character.escape: #FF0000",
+ "light_plus": "constant.character.escape: #EE0000",
"dark_vs": "meta.preprocessor: #569CD6",
"light_vs": "meta.preprocessor: #0000FF",
"hc_black": "constant.character: #569CD6"
@@ -1027,7 +1027,7 @@
"t": "source.cpp meta.preprocessor.macro.cpp meta.block.namespace.cpp meta.body.namespace.cpp constant.character.escape.line-continuation.cpp",
"r": {
"dark_plus": "constant.character.escape: #D7BA7D",
- "light_plus": "constant.character.escape: #FF0000",
+ "light_plus": "constant.character.escape: #EE0000",
"dark_vs": "meta.preprocessor: #569CD6",
"light_vs": "meta.preprocessor: #0000FF",
"hc_black": "constant.character: #569CD6"
@@ -1071,7 +1071,7 @@
"t": "source.cpp meta.preprocessor.macro.cpp constant.character.escape.line-continuation.cpp",
"r": {
"dark_plus": "constant.character.escape: #D7BA7D",
- "light_plus": "constant.character.escape: #FF0000",
+ "light_plus": "constant.character.escape: #EE0000",
"dark_vs": "meta.preprocessor: #569CD6",
"light_vs": "meta.preprocessor: #0000FF",
"hc_black": "constant.character: #569CD6"
diff --git a/extensions/cpp/test/colorize-results/test-80644_cpp.json b/extensions/cpp/test/colorize-results/test-80644_cpp.json
index 580591726a45e..069f31ee72bb6 100644
--- a/extensions/cpp/test/colorize-results/test-80644_cpp.json
+++ b/extensions/cpp/test/colorize-results/test-80644_cpp.json
@@ -378,9 +378,9 @@
"t": "source.cpp meta.function.definition.special.constructor.cpp meta.head.function.definition.special.constructor.cpp meta.parameter.initialization.cpp constant.numeric.decimal.cpp",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -477,9 +477,9 @@
"t": "source.cpp meta.function.definition.special.constructor.cpp meta.head.function.definition.special.constructor.cpp meta.parameter.initialization.cpp constant.numeric.decimal.cpp",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -527,4 +527,4 @@
"hc_black": "default: #FFFFFF"
}
}
-]
\ No newline at end of file
+]
diff --git a/extensions/cpp/test/colorize-results/test-92369_cpp.json b/extensions/cpp/test/colorize-results/test-92369_cpp.json
new file mode 100644
index 0000000000000..fcc9e47435bf6
--- /dev/null
+++ b/extensions/cpp/test/colorize-results/test-92369_cpp.json
@@ -0,0 +1,1927 @@
+[
+ {
+ "c": "std",
+ "t": "source.cpp entity.name.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "tuple_element",
+ "t": "source.cpp entity.name.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "<",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp punctuation.section.angle-brackets.begin.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "0",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp constant.numeric.decimal.cpp",
+ "r": {
+ "dark_plus": "constant.numeric: #B5CEA8",
+ "light_plus": "constant.numeric: #098658",
+ "dark_vs": "constant.numeric: #B5CEA8",
+ "light_vs": "constant.numeric: #098658",
+ "hc_black": "constant.numeric: #B5CEA8"
+ }
+ },
+ {
+ "c": ",",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp punctuation.separator.delimiter.comma.template.argument.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "std",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp entity.name.scope-resolution.template.call.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "pair",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp entity.name.type.cpp",
+ "r": {
+ "dark_plus": "entity.name.type: #4EC9B0",
+ "light_plus": "entity.name.type: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.type: #4EC9B0"
+ }
+ },
+ {
+ "c": "<",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.section.angle-brackets.begin.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "pugi",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp entity.name.scope-resolution.template.call.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "xml_node",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp entity.name.type.cpp",
+ "r": {
+ "dark_plus": "entity.name.type: #4EC9B0",
+ "light_plus": "entity.name.type: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.type: #4EC9B0"
+ }
+ },
+ {
+ "c": ",",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.separator.delimiter.comma.template.argument.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "std",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp entity.name.scope-resolution.template.call.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "map",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp entity.name.type.cpp",
+ "r": {
+ "dark_plus": "entity.name.type: #4EC9B0",
+ "light_plus": "entity.name.type: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.type: #4EC9B0"
+ }
+ },
+ {
+ "c": "<",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.section.angle-brackets.begin.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "std",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp entity.name.scope-resolution.template.call.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "basic_string",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp entity.name.type.cpp",
+ "r": {
+ "dark_plus": "entity.name.type: #4EC9B0",
+ "light_plus": "entity.name.type: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.type: #4EC9B0"
+ }
+ },
+ {
+ "c": "<",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.section.angle-brackets.begin.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "char",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp storage.type.primitive.cpp storage.type.built-in.primitive.cpp",
+ "r": {
+ "dark_plus": "storage.type: #569CD6",
+ "light_plus": "storage.type: #0000FF",
+ "dark_vs": "storage.type: #569CD6",
+ "light_vs": "storage.type: #0000FF",
+ "hc_black": "storage.type: #569CD6"
+ }
+ },
+ {
+ "c": ">",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.section.angle-brackets.end.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": ",",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.separator.delimiter.comma.template.argument.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "pugi",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp entity.name.scope-resolution.template.call.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "xml_node",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp entity.name.type.cpp",
+ "r": {
+ "dark_plus": "entity.name.type: #4EC9B0",
+ "light_plus": "entity.name.type: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.type: #4EC9B0"
+ }
+ },
+ {
+ "c": ",",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.separator.delimiter.comma.template.argument.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "std",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp entity.name.scope-resolution.template.call.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "less",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp entity.name.type.cpp",
+ "r": {
+ "dark_plus": "entity.name.type: #4EC9B0",
+ "light_plus": "entity.name.type: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.type: #4EC9B0"
+ }
+ },
+ {
+ "c": "<",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.section.angle-brackets.begin.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "std",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp entity.name.scope-resolution.template.call.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "basic_string",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp entity.name.type.cpp",
+ "r": {
+ "dark_plus": "entity.name.type: #4EC9B0",
+ "light_plus": "entity.name.type: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.type: #4EC9B0"
+ }
+ },
+ {
+ "c": "<",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.section.angle-brackets.begin.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "char",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp storage.type.primitive.cpp storage.type.built-in.primitive.cpp",
+ "r": {
+ "dark_plus": "storage.type: #569CD6",
+ "light_plus": "storage.type: #0000FF",
+ "dark_vs": "storage.type: #569CD6",
+ "light_vs": "storage.type: #0000FF",
+ "hc_black": "storage.type: #569CD6"
+ }
+ },
+ {
+ "c": ">",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.section.angle-brackets.end.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": ">",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.section.angle-brackets.end.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": ",",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.separator.delimiter.comma.template.argument.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "std",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp entity.name.scope-resolution.template.call.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "allocator",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp entity.name.type.cpp",
+ "r": {
+ "dark_plus": "entity.name.type: #4EC9B0",
+ "light_plus": "entity.name.type: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.type: #4EC9B0"
+ }
+ },
+ {
+ "c": "<",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.section.angle-brackets.begin.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "std",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp entity.name.scope-resolution.template.call.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "pair",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp entity.name.type.cpp",
+ "r": {
+ "dark_plus": "entity.name.type: #4EC9B0",
+ "light_plus": "entity.name.type: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.type: #4EC9B0"
+ }
+ },
+ {
+ "c": "<",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.section.angle-brackets.begin.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "const",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp storage.modifier.specifier.const.cpp",
+ "r": {
+ "dark_plus": "storage.modifier: #569CD6",
+ "light_plus": "storage.modifier: #0000FF",
+ "dark_vs": "storage.modifier: #569CD6",
+ "light_vs": "storage.modifier: #0000FF",
+ "hc_black": "storage.modifier: #569CD6"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "std",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp entity.name.scope-resolution.template.call.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "basic_string",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp entity.name.type.cpp",
+ "r": {
+ "dark_plus": "entity.name.type: #4EC9B0",
+ "light_plus": "entity.name.type: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.type: #4EC9B0"
+ }
+ },
+ {
+ "c": "<",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.section.angle-brackets.begin.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "char",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp storage.type.primitive.cpp storage.type.built-in.primitive.cpp",
+ "r": {
+ "dark_plus": "storage.type: #569CD6",
+ "light_plus": "storage.type: #0000FF",
+ "dark_vs": "storage.type: #569CD6",
+ "light_vs": "storage.type: #0000FF",
+ "hc_black": "storage.type: #569CD6"
+ }
+ },
+ {
+ "c": ">",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.section.angle-brackets.end.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": ",",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.separator.delimiter.comma.template.argument.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "pugi",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp entity.name.scope-resolution.template.call.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "xml_node",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp entity.name.type.cpp",
+ "r": {
+ "dark_plus": "entity.name.type: #4EC9B0",
+ "light_plus": "entity.name.type: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.type: #4EC9B0"
+ }
+ },
+ {
+ "c": ">",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.section.angle-brackets.end.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": ">",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.section.angle-brackets.end.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": ">",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.section.angle-brackets.end.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp meta.qualified_type.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": ">",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp meta.template.call.cpp punctuation.section.angle-brackets.end.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp meta.qualified_type.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": ">",
+ "t": "source.cpp meta.template.call.cpp meta.template.call.cpp punctuation.section.angle-brackets.end.template.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "type dnode",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "std",
+ "t": "source.cpp entity.name.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "_Rb_tree_iterator",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "<",
+ "t": "source.cpp keyword.operator.comparison.cpp",
+ "r": {
+ "dark_plus": "keyword.operator: #D4D4D4",
+ "light_plus": "keyword.operator: #000000",
+ "dark_vs": "keyword.operator: #D4D4D4",
+ "light_vs": "keyword.operator: #000000",
+ "hc_black": "keyword.operator: #D4D4D4"
+ }
+ },
+ {
+ "c": "std",
+ "t": "source.cpp entity.name.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "pair",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "<",
+ "t": "source.cpp keyword.operator.comparison.cpp",
+ "r": {
+ "dark_plus": "keyword.operator: #D4D4D4",
+ "light_plus": "keyword.operator: #000000",
+ "dark_vs": "keyword.operator: #D4D4D4",
+ "light_vs": "keyword.operator: #000000",
+ "hc_black": "keyword.operator: #D4D4D4"
+ }
+ },
+ {
+ "c": "const",
+ "t": "source.cpp storage.modifier.specifier.const.cpp",
+ "r": {
+ "dark_plus": "storage.modifier: #569CD6",
+ "light_plus": "storage.modifier: #0000FF",
+ "dark_vs": "storage.modifier: #569CD6",
+ "light_vs": "storage.modifier: #0000FF",
+ "hc_black": "storage.modifier: #569CD6"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "long",
+ "t": "source.cpp storage.type.primitive.cpp storage.type.built-in.primitive.cpp",
+ "r": {
+ "dark_plus": "storage.type: #569CD6",
+ "light_plus": "storage.type: #0000FF",
+ "dark_vs": "storage.type: #569CD6",
+ "light_vs": "storage.type: #0000FF",
+ "hc_black": "storage.type: #569CD6"
+ }
+ },
+ {
+ "c": ",",
+ "t": "source.cpp punctuation.separator.delimiter.comma.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "std",
+ "t": "source.cpp entity.name.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "pair",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "<",
+ "t": "source.cpp keyword.operator.comparison.cpp",
+ "r": {
+ "dark_plus": "keyword.operator: #D4D4D4",
+ "light_plus": "keyword.operator: #000000",
+ "dark_vs": "keyword.operator: #D4D4D4",
+ "light_vs": "keyword.operator: #000000",
+ "hc_black": "keyword.operator: #D4D4D4"
+ }
+ },
+ {
+ "c": "pugi",
+ "t": "source.cpp entity.name.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "xml_node",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": ",",
+ "t": "source.cpp punctuation.separator.delimiter.comma.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "std",
+ "t": "source.cpp entity.name.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "map",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "<",
+ "t": "source.cpp keyword.operator.comparison.cpp",
+ "r": {
+ "dark_plus": "keyword.operator: #D4D4D4",
+ "light_plus": "keyword.operator: #000000",
+ "dark_vs": "keyword.operator: #D4D4D4",
+ "light_vs": "keyword.operator: #000000",
+ "hc_black": "keyword.operator: #D4D4D4"
+ }
+ },
+ {
+ "c": "std",
+ "t": "source.cpp entity.name.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "basic_string",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "<",
+ "t": "source.cpp keyword.operator.comparison.cpp",
+ "r": {
+ "dark_plus": "keyword.operator: #D4D4D4",
+ "light_plus": "keyword.operator: #000000",
+ "dark_vs": "keyword.operator: #D4D4D4",
+ "light_vs": "keyword.operator: #000000",
+ "hc_black": "keyword.operator: #D4D4D4"
+ }
+ },
+ {
+ "c": "char",
+ "t": "source.cpp storage.type.primitive.cpp storage.type.built-in.primitive.cpp",
+ "r": {
+ "dark_plus": "storage.type: #569CD6",
+ "light_plus": "storage.type: #0000FF",
+ "dark_vs": "storage.type: #569CD6",
+ "light_vs": "storage.type: #0000FF",
+ "hc_black": "storage.type: #569CD6"
+ }
+ },
+ {
+ "c": ">",
+ "t": "source.cpp keyword.operator.comparison.cpp",
+ "r": {
+ "dark_plus": "keyword.operator: #D4D4D4",
+ "light_plus": "keyword.operator: #000000",
+ "dark_vs": "keyword.operator: #D4D4D4",
+ "light_vs": "keyword.operator: #000000",
+ "hc_black": "keyword.operator: #D4D4D4"
+ }
+ },
+ {
+ "c": ",",
+ "t": "source.cpp punctuation.separator.delimiter.comma.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "pugi",
+ "t": "source.cpp entity.name.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "xml_node",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": ",",
+ "t": "source.cpp punctuation.separator.delimiter.comma.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "std",
+ "t": "source.cpp entity.name.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "less",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "<",
+ "t": "source.cpp keyword.operator.comparison.cpp",
+ "r": {
+ "dark_plus": "keyword.operator: #D4D4D4",
+ "light_plus": "keyword.operator: #000000",
+ "dark_vs": "keyword.operator: #D4D4D4",
+ "light_vs": "keyword.operator: #000000",
+ "hc_black": "keyword.operator: #D4D4D4"
+ }
+ },
+ {
+ "c": "std",
+ "t": "source.cpp entity.name.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "basic_string",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "<",
+ "t": "source.cpp keyword.operator.comparison.cpp",
+ "r": {
+ "dark_plus": "keyword.operator: #D4D4D4",
+ "light_plus": "keyword.operator: #000000",
+ "dark_vs": "keyword.operator: #D4D4D4",
+ "light_vs": "keyword.operator: #000000",
+ "hc_black": "keyword.operator: #D4D4D4"
+ }
+ },
+ {
+ "c": "char",
+ "t": "source.cpp storage.type.primitive.cpp storage.type.built-in.primitive.cpp",
+ "r": {
+ "dark_plus": "storage.type: #569CD6",
+ "light_plus": "storage.type: #0000FF",
+ "dark_vs": "storage.type: #569CD6",
+ "light_vs": "storage.type: #0000FF",
+ "hc_black": "storage.type: #569CD6"
+ }
+ },
+ {
+ "c": ">",
+ "t": "source.cpp keyword.operator.comparison.cpp",
+ "r": {
+ "dark_plus": "keyword.operator: #D4D4D4",
+ "light_plus": "keyword.operator: #000000",
+ "dark_vs": "keyword.operator: #D4D4D4",
+ "light_vs": "keyword.operator: #000000",
+ "hc_black": "keyword.operator: #D4D4D4"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": ">",
+ "t": "source.cpp keyword.operator.comparison.cpp",
+ "r": {
+ "dark_plus": "keyword.operator: #D4D4D4",
+ "light_plus": "keyword.operator: #000000",
+ "dark_vs": "keyword.operator: #D4D4D4",
+ "light_vs": "keyword.operator: #000000",
+ "hc_black": "keyword.operator: #D4D4D4"
+ }
+ },
+ {
+ "c": ",",
+ "t": "source.cpp punctuation.separator.delimiter.comma.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "std",
+ "t": "source.cpp entity.name.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "allocator",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "<",
+ "t": "source.cpp keyword.operator.comparison.cpp",
+ "r": {
+ "dark_plus": "keyword.operator: #D4D4D4",
+ "light_plus": "keyword.operator: #000000",
+ "dark_vs": "keyword.operator: #D4D4D4",
+ "light_vs": "keyword.operator: #000000",
+ "hc_black": "keyword.operator: #D4D4D4"
+ }
+ },
+ {
+ "c": "std",
+ "t": "source.cpp entity.name.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "pair",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "<",
+ "t": "source.cpp keyword.operator.comparison.cpp",
+ "r": {
+ "dark_plus": "keyword.operator: #D4D4D4",
+ "light_plus": "keyword.operator: #000000",
+ "dark_vs": "keyword.operator: #D4D4D4",
+ "light_vs": "keyword.operator: #000000",
+ "hc_black": "keyword.operator: #D4D4D4"
+ }
+ },
+ {
+ "c": "const",
+ "t": "source.cpp storage.modifier.specifier.const.cpp",
+ "r": {
+ "dark_plus": "storage.modifier: #569CD6",
+ "light_plus": "storage.modifier: #0000FF",
+ "dark_vs": "storage.modifier: #569CD6",
+ "light_vs": "storage.modifier: #0000FF",
+ "hc_black": "storage.modifier: #569CD6"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "std",
+ "t": "source.cpp entity.name.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "basic_string",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "<",
+ "t": "source.cpp keyword.operator.comparison.cpp",
+ "r": {
+ "dark_plus": "keyword.operator: #D4D4D4",
+ "light_plus": "keyword.operator: #000000",
+ "dark_vs": "keyword.operator: #D4D4D4",
+ "light_vs": "keyword.operator: #000000",
+ "hc_black": "keyword.operator: #D4D4D4"
+ }
+ },
+ {
+ "c": "char",
+ "t": "source.cpp storage.type.primitive.cpp storage.type.built-in.primitive.cpp",
+ "r": {
+ "dark_plus": "storage.type: #569CD6",
+ "light_plus": "storage.type: #0000FF",
+ "dark_vs": "storage.type: #569CD6",
+ "light_vs": "storage.type: #0000FF",
+ "hc_black": "storage.type: #569CD6"
+ }
+ },
+ {
+ "c": ">",
+ "t": "source.cpp keyword.operator.comparison.cpp",
+ "r": {
+ "dark_plus": "keyword.operator: #D4D4D4",
+ "light_plus": "keyword.operator: #000000",
+ "dark_vs": "keyword.operator: #D4D4D4",
+ "light_vs": "keyword.operator: #000000",
+ "hc_black": "keyword.operator: #D4D4D4"
+ }
+ },
+ {
+ "c": ",",
+ "t": "source.cpp punctuation.separator.delimiter.comma.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "pugi",
+ "t": "source.cpp entity.name.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "entity.name.scope-resolution: #4EC9B0",
+ "light_plus": "entity.name.scope-resolution: #267F99",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.scope-resolution: #4EC9B0"
+ }
+ },
+ {
+ "c": "::",
+ "t": "source.cpp punctuation.separator.namespace.access.cpp punctuation.separator.scope-resolution.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "xml_node",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": ">",
+ "t": "source.cpp keyword.operator.comparison.cpp",
+ "r": {
+ "dark_plus": "keyword.operator: #D4D4D4",
+ "light_plus": "keyword.operator: #000000",
+ "dark_vs": "keyword.operator: #D4D4D4",
+ "light_vs": "keyword.operator: #000000",
+ "hc_black": "keyword.operator: #D4D4D4"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": ">",
+ "t": "source.cpp keyword.operator.comparison.cpp",
+ "r": {
+ "dark_plus": "keyword.operator: #D4D4D4",
+ "light_plus": "keyword.operator: #000000",
+ "dark_vs": "keyword.operator: #D4D4D4",
+ "light_vs": "keyword.operator: #000000",
+ "hc_black": "keyword.operator: #D4D4D4"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": ">",
+ "t": "source.cpp keyword.operator.comparison.cpp",
+ "r": {
+ "dark_plus": "keyword.operator: #D4D4D4",
+ "light_plus": "keyword.operator: #000000",
+ "dark_vs": "keyword.operator: #D4D4D4",
+ "light_vs": "keyword.operator: #000000",
+ "hc_black": "keyword.operator: #D4D4D4"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": ">",
+ "t": "source.cpp keyword.operator.comparison.cpp",
+ "r": {
+ "dark_plus": "keyword.operator: #D4D4D4",
+ "light_plus": "keyword.operator: #000000",
+ "dark_vs": "keyword.operator: #D4D4D4",
+ "light_vs": "keyword.operator: #000000",
+ "hc_black": "keyword.operator: #D4D4D4"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": ">",
+ "t": "source.cpp keyword.operator.comparison.cpp",
+ "r": {
+ "dark_plus": "keyword.operator: #D4D4D4",
+ "light_plus": "keyword.operator: #000000",
+ "dark_vs": "keyword.operator: #D4D4D4",
+ "light_vs": "keyword.operator: #000000",
+ "hc_black": "keyword.operator: #D4D4D4"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": ">",
+ "t": "source.cpp keyword.operator.comparison.cpp",
+ "r": {
+ "dark_plus": "keyword.operator: #D4D4D4",
+ "light_plus": "keyword.operator: #000000",
+ "dark_vs": "keyword.operator: #D4D4D4",
+ "light_vs": "keyword.operator: #000000",
+ "hc_black": "keyword.operator: #D4D4D4"
+ }
+ },
+ {
+ "c": " dnode_it ",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "=",
+ "t": "source.cpp keyword.operator.assignment.cpp",
+ "r": {
+ "dark_plus": "keyword.operator: #D4D4D4",
+ "light_plus": "keyword.operator: #000000",
+ "dark_vs": "keyword.operator: #D4D4D4",
+ "light_vs": "keyword.operator: #000000",
+ "hc_black": "keyword.operator: #D4D4D4"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "dnodes_",
+ "t": "source.cpp variable.other.object.access.cpp",
+ "r": {
+ "dark_plus": "variable: #9CDCFE",
+ "light_plus": "variable: #001080",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "variable: #9CDCFE"
+ }
+ },
+ {
+ "c": ".",
+ "t": "source.cpp punctuation.separator.dot-access.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "find",
+ "t": "source.cpp entity.name.function.member.cpp",
+ "r": {
+ "dark_plus": "entity.name.function: #DCDCAA",
+ "light_plus": "entity.name.function: #795E26",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.function: #DCDCAA"
+ }
+ },
+ {
+ "c": "(",
+ "t": "source.cpp punctuation.section.arguments.begin.bracket.round.function.member.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "uid",
+ "t": "source.cpp variable.other.object.access.cpp",
+ "r": {
+ "dark_plus": "variable: #9CDCFE",
+ "light_plus": "variable: #001080",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "variable: #9CDCFE"
+ }
+ },
+ {
+ "c": ".",
+ "t": "source.cpp punctuation.separator.dot-access.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "position",
+ "t": "source.cpp variable.other.property.cpp",
+ "r": {
+ "dark_plus": "variable: #9CDCFE",
+ "light_plus": "variable: #001080",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "variable: #9CDCFE"
+ }
+ },
+ {
+ "c": ")",
+ "t": "source.cpp punctuation.section.arguments.end.bracket.round.function.member.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ }
+]
\ No newline at end of file
diff --git a/extensions/cpp/test/colorize-results/test_c.json b/extensions/cpp/test/colorize-results/test_c.json
index 74be734c5ca9f..30fd5393c476a 100644
--- a/extensions/cpp/test/colorize-results/test_c.json
+++ b/extensions/cpp/test/colorize-results/test_c.json
@@ -851,9 +851,9 @@
"t": "source.c meta.block.c constant.numeric.decimal.c",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -983,9 +983,9 @@
"t": "source.c meta.block.c meta.parens.block.c constant.numeric.decimal.c",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -1181,9 +1181,9 @@
"t": "source.c meta.block.c meta.parens.block.c constant.numeric.decimal.c",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -1390,9 +1390,9 @@
"t": "source.c meta.block.c meta.parens.block.c constant.numeric.decimal.c",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -1720,9 +1720,9 @@
"t": "source.c meta.block.c meta.parens.block.c constant.numeric.decimal.c",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -1863,9 +1863,9 @@
"t": "source.c meta.block.c meta.parens.block.c constant.numeric.decimal.c",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -2226,9 +2226,9 @@
"t": "source.c meta.block.c meta.parens.block.c constant.numeric.decimal.c",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -2391,9 +2391,9 @@
"t": "source.c meta.block.c meta.parens.block.c constant.numeric.decimal.c",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -2765,9 +2765,9 @@
"t": "source.c meta.block.c constant.numeric.decimal.c",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -2793,4 +2793,4 @@
"hc_black": "default: #FFFFFF"
}
}
-]
\ No newline at end of file
+]
diff --git a/extensions/cpp/test/colorize-results/test_cc.json b/extensions/cpp/test/colorize-results/test_cc.json
index 902ef645164b7..324b231bb1bf1 100644
--- a/extensions/cpp/test/colorize-results/test_cc.json
+++ b/extensions/cpp/test/colorize-results/test_cc.json
@@ -36,10 +36,10 @@
"c": "B4G_DEBUG_CHECK",
"t": "source.cpp meta.preprocessor.conditional.cpp entity.name.function.preprocessor.cpp",
"r": {
- "dark_plus": "entity.name.function: #DCDCAA",
- "light_plus": "entity.name.function: #795E26",
- "dark_vs": "meta.preprocessor: #569CD6",
- "light_vs": "meta.preprocessor: #0000FF",
+ "dark_plus": "entity.name.function.preprocessor: #569CD6",
+ "light_plus": "entity.name.function.preprocessor: #0000FF",
+ "dark_vs": "entity.name.function.preprocessor: #569CD6",
+ "light_vs": "entity.name.function.preprocessor: #0000FF",
"hc_black": "entity.name.function: #DCDCAA"
}
},
@@ -268,9 +268,9 @@
"t": "source.cpp meta.parens.cpp constant.numeric.decimal.cpp",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -1807,10 +1807,10 @@
"c": "movw $0x38, %ax; ltr %ax",
"t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp meta.asm.cpp string.quoted.double.cpp meta.embedded.assembly.cpp",
"r": {
- "dark_plus": "meta.embedded: #D4D4D4",
- "light_plus": "meta.embedded: #000000",
- "dark_vs": "meta.embedded: #D4D4D4",
- "light_vs": "meta.embedded: #000000",
+ "dark_plus": "meta.embedded.assembly: #CE9178",
+ "light_plus": "meta.embedded.assembly: #A31515",
+ "dark_vs": "meta.embedded.assembly: #CE9178",
+ "light_vs": "meta.embedded.assembly: #A31515",
"hc_black": "meta.embedded: #FFFFFF"
}
},
@@ -1979,4 +1979,4 @@
"hc_black": "default: #FFFFFF"
}
}
-]
\ No newline at end of file
+]
diff --git a/extensions/cpp/test/colorize-results/test_cpp.json b/extensions/cpp/test/colorize-results/test_cpp.json
index f84d916afa3ab..10f6041bb0004 100644
--- a/extensions/cpp/test/colorize-results/test_cpp.json
+++ b/extensions/cpp/test/colorize-results/test_cpp.json
@@ -190,10 +190,10 @@
"c": "EXTERN_C",
"t": "source.cpp meta.preprocessor.macro.cpp entity.name.function.preprocessor.cpp",
"r": {
- "dark_plus": "entity.name.function: #DCDCAA",
- "light_plus": "entity.name.function: #795E26",
- "dark_vs": "meta.preprocessor: #569CD6",
- "light_vs": "meta.preprocessor: #0000FF",
+ "dark_plus": "entity.name.function.preprocessor: #569CD6",
+ "light_plus": "entity.name.function.preprocessor: #0000FF",
+ "dark_vs": "entity.name.function.preprocessor: #569CD6",
+ "light_vs": "entity.name.function.preprocessor: #0000FF",
"hc_black": "entity.name.function: #DCDCAA"
}
},
@@ -1011,6 +1011,281 @@
"hc_black": "default: #FFFFFF"
}
},
+ {
+ "c": "long",
+ "t": "source.cpp meta.function.definition.special.operator-overload.cpp meta.qualified_type.cpp storage.type.primitive.cpp storage.type.built-in.primitive.cpp",
+ "r": {
+ "dark_plus": "storage.type: #569CD6",
+ "light_plus": "storage.type: #0000FF",
+ "dark_vs": "storage.type: #569CD6",
+ "light_vs": "storage.type: #0000FF",
+ "hc_black": "storage.type: #569CD6"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.function.definition.special.operator-overload.cpp meta.qualified_type.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "double",
+ "t": "source.cpp meta.function.definition.special.operator-overload.cpp meta.qualified_type.cpp storage.type.primitive.cpp storage.type.built-in.primitive.cpp",
+ "r": {
+ "dark_plus": "storage.type: #569CD6",
+ "light_plus": "storage.type: #0000FF",
+ "dark_vs": "storage.type: #569CD6",
+ "light_vs": "storage.type: #0000FF",
+ "hc_black": "storage.type: #569CD6"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.function.definition.special.operator-overload.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "operator",
+ "t": "source.cpp meta.function.definition.special.operator-overload.cpp meta.head.function.definition.special.operator-overload.cpp keyword.other.operator.overload.cpp",
+ "r": {
+ "dark_plus": "keyword.other.operator: #C586C0",
+ "light_plus": "keyword.other.operator: #AF00DB",
+ "dark_vs": "keyword: #569CD6",
+ "light_vs": "keyword: #0000FF",
+ "hc_black": "keyword.other.operator: #C586C0"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.function.definition.special.operator-overload.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "\"\"",
+ "t": "source.cpp meta.function.definition.special.operator-overload.cpp meta.head.function.definition.special.operator-overload.cpp entity.name.operator.custom-literal.cpp",
+ "r": {
+ "dark_plus": "entity.name.operator.custom-literal: #DCDCAA",
+ "light_plus": "entity.name.operator.custom-literal: #795E26",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.function.definition.special.operator-overload.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "_w",
+ "t": "source.cpp meta.function.definition.special.operator-overload.cpp meta.head.function.definition.special.operator-overload.cpp entity.name.operator.custom-literal.cpp",
+ "r": {
+ "dark_plus": "entity.name.operator.custom-literal: #DCDCAA",
+ "light_plus": "entity.name.operator.custom-literal: #795E26",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "(",
+ "t": "source.cpp meta.function.definition.special.operator-overload.cpp meta.head.function.definition.special.operator-overload.cpp punctuation.section.parameters.begin.bracket.round.special.operator-overload.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "long",
+ "t": "source.cpp meta.function.definition.special.operator-overload.cpp meta.head.function.definition.special.operator-overload.cpp meta.function.definition.parameters.special.operator-overload.cpp meta.parameter.cpp storage.type.primitive.cpp storage.type.built-in.primitive.cpp",
+ "r": {
+ "dark_plus": "storage.type: #569CD6",
+ "light_plus": "storage.type: #0000FF",
+ "dark_vs": "storage.type: #569CD6",
+ "light_vs": "storage.type: #0000FF",
+ "hc_black": "storage.type: #569CD6"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.function.definition.special.operator-overload.cpp meta.head.function.definition.special.operator-overload.cpp meta.function.definition.parameters.special.operator-overload.cpp meta.parameter.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "double",
+ "t": "source.cpp meta.function.definition.special.operator-overload.cpp meta.head.function.definition.special.operator-overload.cpp meta.function.definition.parameters.special.operator-overload.cpp meta.parameter.cpp storage.type.primitive.cpp storage.type.built-in.primitive.cpp",
+ "r": {
+ "dark_plus": "storage.type: #569CD6",
+ "light_plus": "storage.type: #0000FF",
+ "dark_vs": "storage.type: #569CD6",
+ "light_vs": "storage.type: #0000FF",
+ "hc_black": "storage.type: #569CD6"
+ }
+ },
+ {
+ "c": ")",
+ "t": "source.cpp meta.function.definition.special.operator-overload.cpp meta.head.function.definition.special.operator-overload.cpp punctuation.section.parameters.end.bracket.round.special.operator-overload.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": ";",
+ "t": "source.cpp punctuation.terminator.statement.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "#",
+ "t": "source.cpp meta.preprocessor.macro.cpp keyword.control.directive.define.cpp punctuation.definition.directive.cpp",
+ "r": {
+ "dark_plus": "keyword.control: #C586C0",
+ "light_plus": "keyword.control: #AF00DB",
+ "dark_vs": "keyword.control: #569CD6",
+ "light_vs": "keyword.control: #0000FF",
+ "hc_black": "keyword.control: #C586C0"
+ }
+ },
+ {
+ "c": "define",
+ "t": "source.cpp meta.preprocessor.macro.cpp keyword.control.directive.define.cpp",
+ "r": {
+ "dark_plus": "keyword.control: #C586C0",
+ "light_plus": "keyword.control: #AF00DB",
+ "dark_vs": "keyword.control: #569CD6",
+ "light_vs": "keyword.control: #0000FF",
+ "hc_black": "keyword.control: #C586C0"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.preprocessor.macro.cpp",
+ "r": {
+ "dark_plus": "meta.preprocessor: #569CD6",
+ "light_plus": "meta.preprocessor: #0000FF",
+ "dark_vs": "meta.preprocessor: #569CD6",
+ "light_vs": "meta.preprocessor: #0000FF",
+ "hc_black": "meta.preprocessor: #569CD6"
+ }
+ },
+ {
+ "c": "MY_MACRO",
+ "t": "source.cpp meta.preprocessor.macro.cpp entity.name.function.preprocessor.cpp",
+ "r": {
+ "dark_plus": "entity.name.function.preprocessor: #569CD6",
+ "light_plus": "entity.name.function.preprocessor: #0000FF",
+ "dark_vs": "entity.name.function.preprocessor: #569CD6",
+ "light_vs": "entity.name.function.preprocessor: #0000FF",
+ "hc_black": "entity.name.function: #DCDCAA"
+ }
+ },
+ {
+ "c": "(",
+ "t": "source.cpp meta.preprocessor.macro.cpp punctuation.definition.parameters.begin.preprocessor.cpp",
+ "r": {
+ "dark_plus": "meta.preprocessor: #569CD6",
+ "light_plus": "meta.preprocessor: #0000FF",
+ "dark_vs": "meta.preprocessor: #569CD6",
+ "light_vs": "meta.preprocessor: #0000FF",
+ "hc_black": "meta.preprocessor: #569CD6"
+ }
+ },
+ {
+ "c": "a",
+ "t": "source.cpp meta.preprocessor.macro.cpp variable.parameter.preprocessor.cpp",
+ "r": {
+ "dark_plus": "variable: #9CDCFE",
+ "light_plus": "variable: #001080",
+ "dark_vs": "meta.preprocessor: #569CD6",
+ "light_vs": "meta.preprocessor: #0000FF",
+ "hc_black": "variable: #9CDCFE"
+ }
+ },
+ {
+ "c": ",",
+ "t": "source.cpp meta.preprocessor.macro.cpp punctuation.separator.parameters.cpp",
+ "r": {
+ "dark_plus": "meta.preprocessor: #569CD6",
+ "light_plus": "meta.preprocessor: #0000FF",
+ "dark_vs": "meta.preprocessor: #569CD6",
+ "light_vs": "meta.preprocessor: #0000FF",
+ "hc_black": "meta.preprocessor: #569CD6"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.preprocessor.macro.cpp",
+ "r": {
+ "dark_plus": "meta.preprocessor: #569CD6",
+ "light_plus": "meta.preprocessor: #0000FF",
+ "dark_vs": "meta.preprocessor: #569CD6",
+ "light_vs": "meta.preprocessor: #0000FF",
+ "hc_black": "meta.preprocessor: #569CD6"
+ }
+ },
+ {
+ "c": "b",
+ "t": "source.cpp meta.preprocessor.macro.cpp variable.parameter.preprocessor.cpp",
+ "r": {
+ "dark_plus": "variable: #9CDCFE",
+ "light_plus": "variable: #001080",
+ "dark_vs": "meta.preprocessor: #569CD6",
+ "light_vs": "meta.preprocessor: #0000FF",
+ "hc_black": "variable: #9CDCFE"
+ }
+ },
+ {
+ "c": ")",
+ "t": "source.cpp meta.preprocessor.macro.cpp punctuation.definition.parameters.end.preprocessor.cpp",
+ "r": {
+ "dark_plus": "meta.preprocessor: #569CD6",
+ "light_plus": "meta.preprocessor: #0000FF",
+ "dark_vs": "meta.preprocessor: #569CD6",
+ "light_vs": "meta.preprocessor: #0000FF",
+ "hc_black": "meta.preprocessor: #569CD6"
+ }
+ },
{
"c": "int",
"t": "source.cpp meta.function.definition.cpp meta.qualified_type.cpp storage.type.primitive.cpp storage.type.built-in.primitive.cpp",
@@ -1099,6 +1374,292 @@
"hc_black": "default: #FFFFFF"
}
},
+ {
+ "c": " ",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "1",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp constant.numeric.decimal.cpp",
+ "r": {
+ "dark_plus": "constant.numeric: #B5CEA8",
+ "light_plus": "constant.numeric: #098658",
+ "dark_vs": "constant.numeric: #B5CEA8",
+ "light_vs": "constant.numeric: #098658",
+ "hc_black": "constant.numeric: #B5CEA8"
+ }
+ },
+ {
+ "c": ".",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp constant.numeric.decimal.point.cpp",
+ "r": {
+ "dark_plus": "constant.numeric: #B5CEA8",
+ "light_plus": "constant.numeric: #098658",
+ "dark_vs": "constant.numeric: #B5CEA8",
+ "light_vs": "constant.numeric: #098658",
+ "hc_black": "constant.numeric: #B5CEA8"
+ }
+ },
+ {
+ "c": "2",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp constant.numeric.decimal.cpp",
+ "r": {
+ "dark_plus": "constant.numeric: #B5CEA8",
+ "light_plus": "constant.numeric: #098658",
+ "dark_vs": "constant.numeric: #B5CEA8",
+ "light_vs": "constant.numeric: #098658",
+ "hc_black": "constant.numeric: #B5CEA8"
+ }
+ },
+ {
+ "c": "_w",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp keyword.other.unit.user-defined.cpp",
+ "r": {
+ "dark_plus": "keyword.other.unit: #B5CEA8",
+ "light_plus": "keyword.other.unit: #098658",
+ "dark_vs": "keyword.other.unit: #B5CEA8",
+ "light_vs": "keyword.other.unit: #098658",
+ "hc_black": "keyword.other.unit: #B5CEA8"
+ }
+ },
+ {
+ "c": ";",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp punctuation.terminator.statement.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp comment.line.double-slash.cpp",
+ "r": {
+ "dark_plus": "comment: #6A9955",
+ "light_plus": "comment: #008000",
+ "dark_vs": "comment: #6A9955",
+ "light_vs": "comment: #008000",
+ "hc_black": "comment: #7CA668"
+ }
+ },
+ {
+ "c": "//",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp comment.line.double-slash.cpp punctuation.definition.comment.cpp",
+ "r": {
+ "dark_plus": "comment: #6A9955",
+ "light_plus": "comment: #008000",
+ "dark_vs": "comment: #6A9955",
+ "light_vs": "comment: #008000",
+ "hc_black": "comment: #7CA668"
+ }
+ },
+ {
+ "c": " calls operator \"\" _w(1.2L)",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp comment.line.double-slash.cpp",
+ "r": {
+ "dark_plus": "comment: #6A9955",
+ "light_plus": "comment: #008000",
+ "dark_vs": "comment: #6A9955",
+ "light_vs": "comment: #008000",
+ "hc_black": "comment: #7CA668"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "asm",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp meta.asm.cpp storage.type.asm.cpp",
+ "r": {
+ "dark_plus": "storage.type: #569CD6",
+ "light_plus": "storage.type: #0000FF",
+ "dark_vs": "storage.type: #569CD6",
+ "light_vs": "storage.type: #0000FF",
+ "hc_black": "storage.type: #569CD6"
+ }
+ },
+ {
+ "c": "(",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp meta.asm.cpp punctuation.section.parens.begin.bracket.round.assembly.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "\"",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp meta.asm.cpp string.quoted.double.cpp punctuation.definition.string.begin.assembly.cpp",
+ "r": {
+ "dark_plus": "string: #CE9178",
+ "light_plus": "string: #A31515",
+ "dark_vs": "string: #CE9178",
+ "light_vs": "string: #A31515",
+ "hc_black": "string: #CE9178"
+ }
+ },
+ {
+ "c": "movl %a %b",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp meta.asm.cpp string.quoted.double.cpp meta.embedded.assembly.cpp",
+ "r": {
+ "dark_plus": "meta.embedded.assembly: #CE9178",
+ "light_plus": "meta.embedded.assembly: #A31515",
+ "dark_vs": "meta.embedded.assembly: #CE9178",
+ "light_vs": "meta.embedded.assembly: #A31515",
+ "hc_black": "meta.embedded: #FFFFFF"
+ }
+ },
+ {
+ "c": "\"",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp meta.asm.cpp string.quoted.double.cpp punctuation.definition.string.end.assembly.cpp",
+ "r": {
+ "dark_plus": "string: #CE9178",
+ "light_plus": "string: #A31515",
+ "dark_vs": "string: #CE9178",
+ "light_vs": "string: #A31515",
+ "hc_black": "string: #CE9178"
+ }
+ },
+ {
+ "c": ")",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp meta.asm.cpp punctuation.section.parens.end.bracket.round.assembly.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": ";",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp punctuation.terminator.statement.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "MY_MACRO",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp entity.name.function.call.cpp",
+ "r": {
+ "dark_plus": "entity.name.function: #DCDCAA",
+ "light_plus": "entity.name.function: #795E26",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "entity.name.function: #DCDCAA"
+ }
+ },
+ {
+ "c": "(",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp punctuation.section.arguments.begin.bracket.round.function.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "1",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp constant.numeric.decimal.cpp",
+ "r": {
+ "dark_plus": "constant.numeric: #B5CEA8",
+ "light_plus": "constant.numeric: #098658",
+ "dark_vs": "constant.numeric: #B5CEA8",
+ "light_vs": "constant.numeric: #098658",
+ "hc_black": "constant.numeric: #B5CEA8"
+ }
+ },
+ {
+ "c": ",",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp punctuation.separator.delimiter.comma.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": " ",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": "2",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp constant.numeric.decimal.cpp",
+ "r": {
+ "dark_plus": "constant.numeric: #B5CEA8",
+ "light_plus": "constant.numeric: #098658",
+ "dark_vs": "constant.numeric: #B5CEA8",
+ "light_vs": "constant.numeric: #098658",
+ "hc_black": "constant.numeric: #B5CEA8"
+ }
+ },
+ {
+ "c": ")",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp punctuation.section.arguments.end.bracket.round.function.call.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
+ {
+ "c": ";",
+ "t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp punctuation.terminator.statement.cpp",
+ "r": {
+ "dark_plus": "default: #D4D4D4",
+ "light_plus": "default: #000000",
+ "dark_vs": "default: #D4D4D4",
+ "light_vs": "default: #000000",
+ "hc_black": "default: #FFFFFF"
+ }
+ },
{
"c": " Rectangle rect",
"t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp",
@@ -1192,9 +1753,9 @@
"t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp constant.numeric.decimal.cpp",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -1214,9 +1775,9 @@
"t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp constant.numeric.decimal.cpp",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -1588,9 +2149,9 @@
"t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp constant.numeric.decimal.cpp",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -1687,9 +2248,9 @@
"t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp meta.parens.cpp constant.numeric.decimal.cpp",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -1753,7 +2314,7 @@
"t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp string.quoted.double.cpp constant.character.escape.cpp",
"r": {
"dark_plus": "constant.character.escape: #D7BA7D",
- "light_plus": "constant.character.escape: #FF0000",
+ "light_plus": "constant.character.escape: #EE0000",
"dark_vs": "string: #CE9178",
"light_vs": "string: #A31515",
"hc_black": "constant.character: #569CD6"
@@ -1841,9 +2402,9 @@
"t": "source.cpp meta.function.definition.cpp meta.body.function.definition.cpp constant.numeric.decimal.cpp",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
diff --git a/extensions/csharp/cgmanifest.json b/extensions/csharp/cgmanifest.json
index beef7f27b8f2c..6156bbb508282 100644
--- a/extensions/csharp/cgmanifest.json
+++ b/extensions/csharp/cgmanifest.json
@@ -6,7 +6,7 @@
"git": {
"name": "dotnet/csharp-tmLanguage",
"repositoryUrl": "https://github.com/dotnet/csharp-tmLanguage",
- "commitHash": "ad7514e8d78542a6ee37f6187091cd4102eb3797"
+ "commitHash": "572697a2c2267430010b3534281f73337144e94f"
}
},
"license": "MIT",
diff --git a/extensions/csharp/package.json b/extensions/csharp/package.json
index 3841eabd13275..74bbb2837883c 100644
--- a/extensions/csharp/package.json
+++ b/extensions/csharp/package.json
@@ -37,7 +37,7 @@
],
"snippets": [{
"language": "csharp",
- "path": "./snippets/csharp.json"
+ "path": "./snippets/csharp.code-snippets"
}]
}
-}
\ No newline at end of file
+}
diff --git a/extensions/csharp/snippets/csharp.json b/extensions/csharp/snippets/csharp.code-snippets
similarity index 100%
rename from extensions/csharp/snippets/csharp.json
rename to extensions/csharp/snippets/csharp.code-snippets
diff --git a/extensions/csharp/syntaxes/csharp.tmLanguage.json b/extensions/csharp/syntaxes/csharp.tmLanguage.json
index 4d80bcc364e5b..8e26aaefa775c 100644
--- a/extensions/csharp/syntaxes/csharp.tmLanguage.json
+++ b/extensions/csharp/syntaxes/csharp.tmLanguage.json
@@ -4,7 +4,7 @@
"If you want to provide a fix or improvement, please create a pull request against the original repository.",
"Once accepted there, we are happy to receive an update request."
],
- "version": "https://github.com/dotnet/csharp-tmLanguage/commit/ad7514e8d78542a6ee37f6187091cd4102eb3797",
+ "version": "https://github.com/dotnet/csharp-tmLanguage/commit/572697a2c2267430010b3534281f73337144e94f",
"name": "C#",
"scopeName": "source.cs",
"patterns": [
@@ -2498,7 +2498,7 @@
},
"interpolation": {
"name": "meta.interpolation.cs",
- "begin": "(?<=[^\\{])((?:\\{\\{)*)(\\{)(?=[^\\{])",
+ "begin": "(?<=[^\\{]|^)((?:\\{\\{)*)(\\{)(?=[^\\{])",
"beginCaptures": {
"1": {
"name": "string.quoted.double.cs"
diff --git a/extensions/csharp/test/colorize-results/test_cs.json b/extensions/csharp/test/colorize-results/test_cs.json
index dbbe61ef3c645..5893af16e9db3 100644
--- a/extensions/csharp/test/colorize-results/test_cs.json
+++ b/extensions/csharp/test/colorize-results/test_cs.json
@@ -444,9 +444,9 @@
"t": "source.cs constant.numeric.decimal.cs",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -477,9 +477,9 @@
"t": "source.cs constant.numeric.decimal.cs",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -510,9 +510,9 @@
"t": "source.cs constant.numeric.decimal.cs",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -543,9 +543,9 @@
"t": "source.cs constant.numeric.decimal.cs",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -576,9 +576,9 @@
"t": "source.cs constant.numeric.decimal.cs",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -719,9 +719,9 @@
"t": "source.cs constant.numeric.decimal.cs",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -1027,9 +1027,9 @@
"t": "source.cs constant.numeric.decimal.cs",
"r": {
"dark_plus": "constant.numeric: #B5CEA8",
- "light_plus": "constant.numeric: #09885A",
+ "light_plus": "constant.numeric: #098658",
"dark_vs": "constant.numeric: #B5CEA8",
- "light_vs": "constant.numeric: #09885A",
+ "light_vs": "constant.numeric: #098658",
"hc_black": "constant.numeric: #B5CEA8"
}
},
@@ -1374,4 +1374,4 @@
"hc_black": "default: #FFFFFF"
}
}
-]
\ No newline at end of file
+]
diff --git a/extensions/css-language-features/.vscodeignore b/extensions/css-language-features/.vscodeignore
index fa38a47136231..a08d9b8dec775 100644
--- a/extensions/css-language-features/.vscodeignore
+++ b/extensions/css-language-features/.vscodeignore
@@ -16,4 +16,6 @@ server/.npmignore
yarn.lock
server/extension.webpack.config.js
extension.webpack.config.js
-CONTRIBUTING.md
\ No newline at end of file
+server/extension-browser.webpack.config.js
+extension-browser.webpack.config.js
+CONTRIBUTING.md
diff --git a/extensions/css-language-features/client/src/browser/cssClientMain.ts b/extensions/css-language-features/client/src/browser/cssClientMain.ts
new file mode 100644
index 0000000000000..2a5e3e1f2c24e
--- /dev/null
+++ b/extensions/css-language-features/client/src/browser/cssClientMain.ts
@@ -0,0 +1,32 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import { ExtensionContext, Uri } from 'vscode';
+import { LanguageClientOptions } from 'vscode-languageclient';
+import { startClient, LanguageClientConstructor } from '../cssClient';
+import { LanguageClient } from 'vscode-languageclient/browser';
+
+declare const Worker: {
+ new(stringUrl: string): any;
+};
+declare const TextDecoder: {
+ new(encoding?: string): { decode(buffer: ArrayBuffer): string; };
+};
+
+// this method is called when vs code is activated
+export function activate(context: ExtensionContext) {
+ const serverMain = Uri.joinPath(context.extensionUri, 'server/dist/browser/cssServerMain.js');
+ try {
+ const worker = new Worker(serverMain.toString());
+ const newLanguageClient: LanguageClientConstructor = (id: string, name: string, clientOptions: LanguageClientOptions) => {
+ return new LanguageClient(id, name, clientOptions, worker);
+ };
+
+ startClient(context, newLanguageClient, { TextDecoder });
+
+ } catch (e) {
+ console.log(e);
+ }
+}
diff --git a/extensions/css-language-features/client/src/cssClient.ts b/extensions/css-language-features/client/src/cssClient.ts
new file mode 100644
index 0000000000000..4bfa95c843987
--- /dev/null
+++ b/extensions/css-language-features/client/src/cssClient.ts
@@ -0,0 +1,166 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import { commands, CompletionItem, CompletionItemKind, ExtensionContext, languages, Position, Range, SnippetString, TextEdit, window, TextDocument, CompletionContext, CancellationToken, ProviderResult, CompletionList } from 'vscode';
+import { Disposable, LanguageClientOptions, ProvideCompletionItemsSignature, NotificationType, CommonLanguageClient } from 'vscode-languageclient';
+import * as nls from 'vscode-nls';
+import { getCustomDataSource } from './customData';
+import { RequestService, serveFileSystemRequests } from './requests';
+
+namespace CustomDataChangedNotification {
+ export const type: NotificationType = new NotificationType('css/customDataChanged');
+}
+
+const localize = nls.loadMessageBundle();
+
+export type LanguageClientConstructor = (name: string, description: string, clientOptions: LanguageClientOptions) => CommonLanguageClient;
+
+export interface Runtime {
+ TextDecoder: { new(encoding?: string): { decode(buffer: ArrayBuffer): string; } };
+ fs?: RequestService;
+}
+
+export function startClient(context: ExtensionContext, newLanguageClient: LanguageClientConstructor, runtime: Runtime) {
+
+ const customDataSource = getCustomDataSource(context.subscriptions);
+
+ let documentSelector = ['css', 'scss', 'less'];
+
+ // Options to control the language client
+ let clientOptions: LanguageClientOptions = {
+ documentSelector,
+ synchronize: {
+ configurationSection: ['css', 'scss', 'less']
+ },
+ initializationOptions: {
+ handledSchemas: ['file']
+ },
+ middleware: {
+ provideCompletionItem(document: TextDocument, position: Position, context: CompletionContext, token: CancellationToken, next: ProvideCompletionItemsSignature): ProviderResult {
+ // testing the replace / insert mode
+ function updateRanges(item: CompletionItem) {
+ const range = item.range;
+ if (range instanceof Range && range.end.isAfter(position) && range.start.isBeforeOrEqual(position)) {
+ item.range = { inserting: new Range(range.start, position), replacing: range };
+
+ }
+ }
+ function updateLabel(item: CompletionItem) {
+ if (item.kind === CompletionItemKind.Color) {
+ item.label2 = {
+ name: item.label,
+ type: (item.documentation as string)
+ };
+ }
+ }
+ // testing the new completion
+ function updateProposals(r: CompletionItem[] | CompletionList | null | undefined): CompletionItem[] | CompletionList | null | undefined {
+ if (r) {
+ (Array.isArray(r) ? r : r.items).forEach(updateRanges);
+ (Array.isArray(r) ? r : r.items).forEach(updateLabel);
+ }
+ return r;
+ }
+ const isThenable = (obj: ProviderResult): obj is Thenable => obj && (obj)['then'];
+
+ const r = next(document, position, context, token);
+ if (isThenable(r)) {
+ return r.then(updateProposals);
+ }
+ return updateProposals(r);
+ }
+ }
+ };
+
+ // Create the language client and start the client.
+ let client = newLanguageClient('css', localize('cssserver.name', 'CSS Language Server'), clientOptions);
+ client.registerProposedFeatures();
+ client.onReady().then(() => {
+
+ client.sendNotification(CustomDataChangedNotification.type, customDataSource.uris);
+ customDataSource.onDidChange(() => {
+ client.sendNotification(CustomDataChangedNotification.type, customDataSource.uris);
+ });
+
+ serveFileSystemRequests(client, runtime);
+ });
+
+ let disposable = client.start();
+ // Push the disposable to the context's subscriptions so that the
+ // client can be deactivated on extension deactivation
+ context.subscriptions.push(disposable);
+
+ let indentationRules = {
+ increaseIndentPattern: /(^.*\{[^}]*$)/,
+ decreaseIndentPattern: /^\s*\}/
+ };
+
+ languages.setLanguageConfiguration('css', {
+ wordPattern: /(#?-?\d*\.\d\w*%?)|(::?[\w-]*(?=[^,{;]*[,{]))|(([@#.!])?[\w-?]+%?|[@#!.])/g,
+ indentationRules: indentationRules
+ });
+
+ languages.setLanguageConfiguration('less', {
+ wordPattern: /(#?-?\d*\.\d\w*%?)|(::?[\w-]+(?=[^,{;]*[,{]))|(([@#.!])?[\w-?]+%?|[@#!.])/g,
+ indentationRules: indentationRules
+ });
+
+ languages.setLanguageConfiguration('scss', {
+ wordPattern: /(#?-?\d*\.\d\w*%?)|(::?[\w-]*(?=[^,{;]*[,{]))|(([@$#.!])?[\w-?]+%?|[@#!$.])/g,
+ indentationRules: indentationRules
+ });
+
+ client.onReady().then(() => {
+ context.subscriptions.push(initCompletionProvider());
+ });
+
+ function initCompletionProvider(): Disposable {
+ const regionCompletionRegExpr = /^(\s*)(\/(\*\s*(#\w*)?)?)?$/;
+
+ return languages.registerCompletionItemProvider(documentSelector, {
+ provideCompletionItems(doc: TextDocument, pos: Position) {
+ let lineUntilPos = doc.getText(new Range(new Position(pos.line, 0), pos));
+ let match = lineUntilPos.match(regionCompletionRegExpr);
+ if (match) {
+ let range = new Range(new Position(pos.line, match[1].length), pos);
+ let beginProposal = new CompletionItem('#region', CompletionItemKind.Snippet);
+ beginProposal.range = range; TextEdit.replace(range, '/* #region */');
+ beginProposal.insertText = new SnippetString('/* #region $1*/');
+ beginProposal.documentation = localize('folding.start', 'Folding Region Start');
+ beginProposal.filterText = match[2];
+ beginProposal.sortText = 'za';
+ let endProposal = new CompletionItem('#endregion', CompletionItemKind.Snippet);
+ endProposal.range = range;
+ endProposal.insertText = '/* #endregion */';
+ endProposal.documentation = localize('folding.end', 'Folding Region End');
+ endProposal.sortText = 'zb';
+ endProposal.filterText = match[2];
+ return [beginProposal, endProposal];
+ }
+ return null;
+ }
+ });
+ }
+
+ commands.registerCommand('_css.applyCodeAction', applyCodeAction);
+
+ function applyCodeAction(uri: string, documentVersion: number, edits: TextEdit[]) {
+ let textEditor = window.activeTextEditor;
+ if (textEditor && textEditor.document.uri.toString() === uri) {
+ if (textEditor.document.version !== documentVersion) {
+ window.showInformationMessage(`CSS fix is outdated and can't be applied to the document.`);
+ }
+ textEditor.edit(mutator => {
+ for (let edit of edits) {
+ mutator.replace(client.protocol2CodeConverter.asRange(edit.range), edit.newText);
+ }
+ }).then(success => {
+ if (!success) {
+ window.showErrorMessage('Failed to apply CSS fix to the document. Please consider opening an issue with steps to reproduce.');
+ }
+ });
+ }
+ }
+}
diff --git a/extensions/css-language-features/client/src/cssMain.ts b/extensions/css-language-features/client/src/cssMain.ts
deleted file mode 100644
index 1bc18bf634628..0000000000000
--- a/extensions/css-language-features/client/src/cssMain.ts
+++ /dev/null
@@ -1,139 +0,0 @@
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-
-import * as fs from 'fs';
-import * as path from 'path';
-import { commands, CompletionItem, CompletionItemKind, ExtensionContext, languages, Position, Range, SnippetString, TextEdit, window, workspace } from 'vscode';
-import { Disposable, LanguageClient, LanguageClientOptions, ServerOptions, TransportKind } from 'vscode-languageclient';
-import * as nls from 'vscode-nls';
-import { getCustomDataPathsFromAllExtensions, getCustomDataPathsInAllWorkspaces } from './customData';
-
-const localize = nls.loadMessageBundle();
-
-// this method is called when vs code is activated
-export function activate(context: ExtensionContext) {
-
- let serverMain = readJSONFile(context.asAbsolutePath('./server/package.json')).main;
- let serverModule = context.asAbsolutePath(path.join('server', serverMain));
-
- // The debug options for the server
- let debugOptions = { execArgv: ['--nolazy', '--inspect=6044'] };
-
- // If the extension is launch in debug mode the debug server options are use
- // Otherwise the run options are used
- let serverOptions: ServerOptions = {
- run: { module: serverModule, transport: TransportKind.ipc },
- debug: { module: serverModule, transport: TransportKind.ipc, options: debugOptions }
- };
-
- let documentSelector = ['css', 'scss', 'less'];
-
- let dataPaths = [
- ...getCustomDataPathsInAllWorkspaces(workspace.workspaceFolders),
- ...getCustomDataPathsFromAllExtensions()
- ];
-
- // Options to control the language client
- let clientOptions: LanguageClientOptions = {
- documentSelector,
- synchronize: {
- configurationSection: ['css', 'scss', 'less']
- },
- initializationOptions: {
- dataPaths
- }
- };
-
- // Create the language client and start the client.
- let client = new LanguageClient('css', localize('cssserver.name', 'CSS Language Server'), serverOptions, clientOptions);
- client.registerProposedFeatures();
-
- let disposable = client.start();
- // Push the disposable to the context's subscriptions so that the
- // client can be deactivated on extension deactivation
- context.subscriptions.push(disposable);
-
- let indentationRules = {
- increaseIndentPattern: /(^.*\{[^}]*$)/,
- decreaseIndentPattern: /^\s*\}/
- };
-
- languages.setLanguageConfiguration('css', {
- wordPattern: /(#?-?\d*\.\d\w*%?)|(::?[\w-]*(?=[^,{;]*[,{]))|(([@#.!])?[\w-?]+%?|[@#!.])/g,
- indentationRules: indentationRules
- });
-
- languages.setLanguageConfiguration('less', {
- wordPattern: /(#?-?\d*\.\d\w*%?)|(::?[\w-]+(?=[^,{;]*[,{]))|(([@#.!])?[\w-?]+%?|[@#!.])/g,
- indentationRules: indentationRules
- });
-
- languages.setLanguageConfiguration('scss', {
- wordPattern: /(#?-?\d*\.\d\w*%?)|(::?[\w-]*(?=[^,{;]*[,{]))|(([@$#.!])?[\w-?]+%?|[@#!$.])/g,
- indentationRules: indentationRules
- });
-
- client.onReady().then(() => {
- context.subscriptions.push(initCompletionProvider());
- });
-
- function initCompletionProvider(): Disposable {
- const regionCompletionRegExpr = /^(\s*)(\/(\*\s*(#\w*)?)?)?$/;
-
- return languages.registerCompletionItemProvider(documentSelector, {
- provideCompletionItems(doc, pos) {
- let lineUntilPos = doc.getText(new Range(new Position(pos.line, 0), pos));
- let match = lineUntilPos.match(regionCompletionRegExpr);
- if (match) {
- let range = new Range(new Position(pos.line, match[1].length), pos);
- let beginProposal = new CompletionItem('#region', CompletionItemKind.Snippet);
- beginProposal.range = range; TextEdit.replace(range, '/* #region */');
- beginProposal.insertText = new SnippetString('/* #region $1*/');
- beginProposal.documentation = localize('folding.start', 'Folding Region Start');
- beginProposal.filterText = match[2];
- beginProposal.sortText = 'za';
- let endProposal = new CompletionItem('#endregion', CompletionItemKind.Snippet);
- endProposal.range = range;
- endProposal.insertText = '/* #endregion */';
- endProposal.documentation = localize('folding.end', 'Folding Region End');
- endProposal.sortText = 'zb';
- endProposal.filterText = match[2];
- return [beginProposal, endProposal];
- }
- return null;
- }
- });
- }
-
- commands.registerCommand('_css.applyCodeAction', applyCodeAction);
-
- function applyCodeAction(uri: string, documentVersion: number, edits: TextEdit[]) {
- let textEditor = window.activeTextEditor;
- if (textEditor && textEditor.document.uri.toString() === uri) {
- if (textEditor.document.version !== documentVersion) {
- window.showInformationMessage(`CSS fix is outdated and can't be applied to the document.`);
- }
- textEditor.edit(mutator => {
- for (let edit of edits) {
- mutator.replace(client.protocol2CodeConverter.asRange(edit.range), edit.newText);
- }
- }).then(success => {
- if (!success) {
- window.showErrorMessage('Failed to apply CSS fix to the document. Please consider opening an issue with steps to reproduce.');
- }
- });
- }
- }
-}
-
-function readJSONFile(location: string) {
- try {
- return JSON.parse(fs.readFileSync(location).toString());
- } catch (e) {
- console.log(`Problems reading ${location}: ${e}`);
- return {};
- }
-}
-
diff --git a/extensions/css-language-features/client/src/customData.ts b/extensions/css-language-features/client/src/customData.ts
index 7054d0f1ba96d..24b56f12b0612 100644
--- a/extensions/css-language-features/client/src/customData.ts
+++ b/extensions/css-language-features/client/src/customData.ts
@@ -3,54 +3,86 @@
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
-import * as path from 'path';
-import { workspace, WorkspaceFolder, extensions } from 'vscode';
+import { workspace, extensions, Uri, EventEmitter, Disposable } from 'vscode';
+import { resolvePath, joinPath } from './requests';
-interface ExperimentalConfig {
- customData?: string[];
- experimental?: {
- customData?: string[];
+export function getCustomDataSource(toDispose: Disposable[]) {
+ let pathsInWorkspace = getCustomDataPathsInAllWorkspaces();
+ let pathsInExtensions = getCustomDataPathsFromAllExtensions();
+
+ const onChange = new EventEmitter();
+
+ toDispose.push(extensions.onDidChange(_ => {
+ const newPathsInExtensions = getCustomDataPathsFromAllExtensions();
+ if (newPathsInExtensions.length !== pathsInExtensions.length || !newPathsInExtensions.every((val, idx) => val === pathsInExtensions[idx])) {
+ pathsInExtensions = newPathsInExtensions;
+ onChange.fire();
+ }
+ }));
+ toDispose.push(workspace.onDidChangeConfiguration(e => {
+ if (e.affectsConfiguration('css.customData')) {
+ pathsInWorkspace = getCustomDataPathsInAllWorkspaces();
+ onChange.fire();
+ }
+ }));
+
+ return {
+ get uris() {
+ return pathsInWorkspace.concat(pathsInExtensions);
+ },
+ get onDidChange() {
+ return onChange.event;
+ }
};
}
-export function getCustomDataPathsInAllWorkspaces(workspaceFolders: WorkspaceFolder[] | undefined): string[] {
+
+function getCustomDataPathsInAllWorkspaces(): string[] {
+ const workspaceFolders = workspace.workspaceFolders;
+
const dataPaths: string[] = [];
if (!workspaceFolders) {
return dataPaths;
}
- workspaceFolders.forEach(wf => {
- const allCssConfig = workspace.getConfiguration(undefined, wf.uri);
- const wfCSSConfig = allCssConfig.inspect('css');
- if (wfCSSConfig && wfCSSConfig.workspaceFolderValue && wfCSSConfig.workspaceFolderValue.customData) {
- const customData = wfCSSConfig.workspaceFolderValue.customData;
- if (Array.isArray(customData)) {
- customData.forEach(t => {
- if (typeof t === 'string') {
- dataPaths.push(path.resolve(wf.uri.fsPath, t));
- }
- });
+ const collect = (paths: string[] | undefined, rootFolder: Uri) => {
+ if (Array.isArray(paths)) {
+ for (const path of paths) {
+ if (typeof path === 'string') {
+ dataPaths.push(resolvePath(rootFolder, path).toString());
+ }
}
}
- });
+ };
+ for (let i = 0; i < workspaceFolders.length; i++) {
+ const folderUri = workspaceFolders[i].uri;
+ const allCssConfig = workspace.getConfiguration('css', folderUri);
+ const customDataInspect = allCssConfig.inspect('customData');
+ if (customDataInspect) {
+ collect(customDataInspect.workspaceFolderValue, folderUri);
+ if (i === 0) {
+ if (workspace.workspaceFile) {
+ collect(customDataInspect.workspaceValue, workspace.workspaceFile);
+ }
+ collect(customDataInspect.globalValue, folderUri);
+ }
+ }
+
+ }
return dataPaths;
}
-export function getCustomDataPathsFromAllExtensions(): string[] {
+function getCustomDataPathsFromAllExtensions(): string[] {
const dataPaths: string[] = [];
-
for (const extension of extensions.all) {
- const contributes = extension.packageJSON && extension.packageJSON.contributes;
-
- if (contributes && contributes.css && contributes.css.customData && Array.isArray(contributes.css.customData)) {
- const relativePaths: string[] = contributes.css.customData;
- relativePaths.forEach(rp => {
- dataPaths.push(path.resolve(extension.extensionPath, rp));
- });
+ const customData = extension.packageJSON?.contributes?.css?.customData;
+ if (Array.isArray(customData)) {
+ for (const rp of customData) {
+ dataPaths.push(joinPath(extension.extensionUri, rp).toString());
+ }
}
}
-
return dataPaths;
}
diff --git a/extensions/css-language-features/client/src/node/cssClientMain.ts b/extensions/css-language-features/client/src/node/cssClientMain.ts
new file mode 100644
index 0000000000000..cc675b494c2b3
--- /dev/null
+++ b/extensions/css-language-features/client/src/node/cssClientMain.ts
@@ -0,0 +1,35 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import { getNodeFSRequestService } from './nodeFs';
+import { ExtensionContext, extensions } from 'vscode';
+import { startClient, LanguageClientConstructor } from '../cssClient';
+import { ServerOptions, TransportKind, LanguageClientOptions, LanguageClient } from 'vscode-languageclient/node';
+import { TextDecoder } from 'util';
+
+// this method is called when vs code is activated
+export function activate(context: ExtensionContext) {
+
+ const clientMain = extensions.getExtension('vscode.css-language-features')?.packageJSON?.main || '';
+
+ const serverMain = `./server/${clientMain.indexOf('/dist/') !== -1 ? 'dist' : 'out'}/node/cssServerMain`;
+ const serverModule = context.asAbsolutePath(serverMain);
+
+ // The debug options for the server
+ const debugOptions = { execArgv: ['--nolazy', '--inspect=6044'] };
+
+ // If the extension is launch in debug mode the debug server options are use
+ // Otherwise the run options are used
+ const serverOptions: ServerOptions = {
+ run: { module: serverModule, transport: TransportKind.ipc },
+ debug: { module: serverModule, transport: TransportKind.ipc, options: debugOptions }
+ };
+
+ const newLanguageClient: LanguageClientConstructor = (id: string, name: string, clientOptions: LanguageClientOptions) => {
+ return new LanguageClient(id, name, serverOptions, clientOptions);
+ };
+
+ startClient(context, newLanguageClient, { fs: getNodeFSRequestService(), TextDecoder });
+}
diff --git a/extensions/css-language-features/client/src/node/nodeFs.ts b/extensions/css-language-features/client/src/node/nodeFs.ts
new file mode 100644
index 0000000000000..c13ef2e1c08d5
--- /dev/null
+++ b/extensions/css-language-features/client/src/node/nodeFs.ts
@@ -0,0 +1,85 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import * as fs from 'fs';
+import { Uri } from 'vscode';
+import { getScheme, RequestService, FileType } from '../requests';
+
+export function getNodeFSRequestService(): RequestService {
+ function ensureFileUri(location: string) {
+ if (getScheme(location) !== 'file') {
+ throw new Error('fileRequestService can only handle file URLs');
+ }
+ }
+ return {
+ getContent(location: string, encoding?: string) {
+ ensureFileUri(location);
+ return new Promise((c, e) => {
+ const uri = Uri.parse(location);
+ fs.readFile(uri.fsPath, encoding, (err, buf) => {
+ if (err) {
+ return e(err);
+ }
+ c(buf.toString());
+
+ });
+ });
+ },
+ stat(location: string) {
+ ensureFileUri(location);
+ return new Promise((c, e) => {
+ const uri = Uri.parse(location);
+ fs.stat(uri.fsPath, (err, stats) => {
+ if (err) {
+ if (err.code === 'ENOENT') {
+ return c({ type: FileType.Unknown, ctime: -1, mtime: -1, size: -1 });
+ } else {
+ return e(err);
+ }
+ }
+
+ let type = FileType.Unknown;
+ if (stats.isFile()) {
+ type = FileType.File;
+ } else if (stats.isDirectory()) {
+ type = FileType.Directory;
+ } else if (stats.isSymbolicLink()) {
+ type = FileType.SymbolicLink;
+ }
+
+ c({
+ type,
+ ctime: stats.ctime.getTime(),
+ mtime: stats.mtime.getTime(),
+ size: stats.size
+ });
+ });
+ });
+ },
+ readDirectory(location: string) {
+ ensureFileUri(location);
+ return new Promise((c, e) => {
+ const path = Uri.parse(location).fsPath;
+
+ fs.readdir(path, { withFileTypes: true }, (err, children) => {
+ if (err) {
+ return e(err);
+ }
+ c(children.map(stat => {
+ if (stat.isSymbolicLink()) {
+ return [stat.name, FileType.SymbolicLink];
+ } else if (stat.isDirectory()) {
+ return [stat.name, FileType.Directory];
+ } else if (stat.isFile()) {
+ return [stat.name, FileType.File];
+ } else {
+ return [stat.name, FileType.Unknown];
+ }
+ }));
+ });
+ });
+ }
+ };
+}
diff --git a/extensions/css-language-features/client/src/requests.ts b/extensions/css-language-features/client/src/requests.ts
new file mode 100644
index 0000000000000..1b1e70b2d882b
--- /dev/null
+++ b/extensions/css-language-features/client/src/requests.ts
@@ -0,0 +1,148 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import { Uri, workspace } from 'vscode';
+import { RequestType, CommonLanguageClient } from 'vscode-languageclient';
+import { Runtime } from './cssClient';
+
+export namespace FsContentRequest {
+ export const type: RequestType<{ uri: string; encoding?: string; }, string, any, any> = new RequestType('fs/content');
+}
+export namespace FsStatRequest {
+ export const type: RequestType = new RequestType('fs/stat');
+}
+
+export namespace FsReadDirRequest {
+ export const type: RequestType = new RequestType('fs/readDir');
+}
+
+export function serveFileSystemRequests(client: CommonLanguageClient, runtime: Runtime) {
+ client.onRequest(FsContentRequest.type, (param: { uri: string; encoding?: string; }) => {
+ const uri = Uri.parse(param.uri);
+ if (uri.scheme === 'file' && runtime.fs) {
+ return runtime.fs.getContent(param.uri);
+ }
+ return workspace.fs.readFile(uri).then(buffer => {
+ return new runtime.TextDecoder(param.encoding).decode(buffer);
+ });
+ });
+ client.onRequest(FsReadDirRequest.type, (uriString: string) => {
+ const uri = Uri.parse(uriString);
+ if (uri.scheme === 'file' && runtime.fs) {
+ return runtime.fs.readDirectory(uriString);
+ }
+ return workspace.fs.readDirectory(uri);
+ });
+ client.onRequest(FsStatRequest.type, (uriString: string) => {
+ const uri = Uri.parse(uriString);
+ if (uri.scheme === 'file' && runtime.fs) {
+ return runtime.fs.stat(uriString);
+ }
+ return workspace.fs.stat(uri);
+ });
+}
+
+export enum FileType {
+ /**
+ * The file type is unknown.
+ */
+ Unknown = 0,
+ /**
+ * A regular file.
+ */
+ File = 1,
+ /**
+ * A directory.
+ */
+ Directory = 2,
+ /**
+ * A symbolic link to a file.
+ */
+ SymbolicLink = 64
+}
+export interface FileStat {
+ /**
+ * The type of the file, e.g. is a regular file, a directory, or symbolic link
+ * to a file.
+ */
+ type: FileType;
+ /**
+ * The creation timestamp in milliseconds elapsed since January 1, 1970 00:00:00 UTC.
+ */
+ ctime: number;
+ /**
+ * The modification timestamp in milliseconds elapsed since January 1, 1970 00:00:00 UTC.
+ */
+ mtime: number;
+ /**
+ * The size in bytes.
+ */
+ size: number;
+}
+
+export interface RequestService {
+ getContent(uri: string, encoding?: string): Promise;
+
+ stat(uri: string): Promise;
+ readDirectory(uri: string): Promise<[string, FileType][]>;
+}
+
+export function getScheme(uri: string) {
+ return uri.substr(0, uri.indexOf(':'));
+}
+
+export function dirname(uri: string) {
+ const lastIndexOfSlash = uri.lastIndexOf('/');
+ return lastIndexOfSlash !== -1 ? uri.substr(0, lastIndexOfSlash) : '';
+}
+
+export function basename(uri: string) {
+ const lastIndexOfSlash = uri.lastIndexOf('/');
+ return uri.substr(lastIndexOfSlash + 1);
+}
+
+const Slash = '/'.charCodeAt(0);
+const Dot = '.'.charCodeAt(0);
+
+export function isAbsolutePath(path: string) {
+ return path.charCodeAt(0) === Slash;
+}
+
+export function resolvePath(uri: Uri, path: string): Uri {
+ if (isAbsolutePath(path)) {
+ return uri.with({ path: normalizePath(path.split('/')) });
+ }
+ return joinPath(uri, path);
+}
+
+export function normalizePath(parts: string[]): string {
+ const newParts: string[] = [];
+ for (const part of parts) {
+ if (part.length === 0 || part.length === 1 && part.charCodeAt(0) === Dot) {
+ // ignore
+ } else if (part.length === 2 && part.charCodeAt(0) === Dot && part.charCodeAt(1) === Dot) {
+ newParts.pop();
+ } else {
+ newParts.push(part);
+ }
+ }
+ if (parts.length > 1 && parts[parts.length - 1].length === 0) {
+ newParts.push('');
+ }
+ let res = newParts.join('/');
+ if (parts[0].length === 0) {
+ res = '/' + res;
+ }
+ return res;
+}
+
+
+export function joinPath(uri: Uri, ...paths: string[]): Uri {
+ const parts = uri.path.split('/');
+ for (let path of paths) {
+ parts.push(...path.split('/'));
+ }
+ return uri.with({ path: normalizePath(parts) });
+}
diff --git a/extensions/css-language-features/extension-browser.webpack.config.js b/extensions/css-language-features/extension-browser.webpack.config.js
new file mode 100644
index 0000000000000..cb2e13c7ed316
--- /dev/null
+++ b/extensions/css-language-features/extension-browser.webpack.config.js
@@ -0,0 +1,22 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+//@ts-check
+
+'use strict';
+
+const withBrowserDefaults = require('../shared.webpack.config').browser;
+const path = require('path');
+
+module.exports = withBrowserDefaults({
+ context: path.join(__dirname, 'client'),
+ entry: {
+ extension: './src/browser/cssClientMain.ts'
+ },
+ output: {
+ filename: 'cssClientMain.js',
+ path: path.join(__dirname, 'client', 'dist', 'browser')
+ }
+});
diff --git a/extensions/css-language-features/extension.webpack.config.js b/extensions/css-language-features/extension.webpack.config.js
index dec7ad5afb4a0..a931210ab32ea 100644
--- a/extensions/css-language-features/extension.webpack.config.js
+++ b/extensions/css-language-features/extension.webpack.config.js
@@ -13,10 +13,10 @@ const path = require('path');
module.exports = withDefaults({
context: path.join(__dirname, 'client'),
entry: {
- extension: './src/cssMain.ts',
+ extension: './src/node/cssClientMain.ts',
},
output: {
- filename: 'cssMain.js',
- path: path.join(__dirname, 'client', 'dist')
+ filename: 'cssClientMain.js',
+ path: path.join(__dirname, 'client', 'dist', 'node')
}
});
diff --git a/extensions/css-language-features/package.json b/extensions/css-language-features/package.json
index 702d9d755cad0..0b632903b52ff 100644
--- a/extensions/css-language-features/package.json
+++ b/extensions/css-language-features/package.json
@@ -15,7 +15,8 @@
"onLanguage:scss",
"onCommand:_css.applyCodeAction"
],
- "main": "./client/out/cssMain",
+ "main": "./client/out/node/cssClientMain",
+ "browser": "./client/dist/browser/cssClientMain",
"enableProposedApi": true,
"scripts": {
"compile": "gulp compile-extension:css-language-features-client compile-extension:css-language-features-server",
@@ -783,6 +784,17 @@
}
}
],
+ "configurationDefaults": {
+ "[css]": {
+ "editor.suggest.insertMode": "replace"
+ },
+ "[scss]": {
+ "editor.suggest.insertMode": "replace"
+ },
+ "[less]": {
+ "editor.suggest.insertMode": "replace"
+ }
+ },
"jsonValidation": [
{
"fileMatch": "*.css-data.json",
@@ -795,11 +807,11 @@
]
},
"dependencies": {
- "vscode-languageclient": "^6.0.0-next.3",
- "vscode-nls": "^4.1.1"
+ "vscode-languageclient": "7.0.0-next.5.1",
+ "vscode-nls": "^4.1.2"
},
"devDependencies": {
"@types/node": "^12.11.7",
- "mocha": "^6.1.4"
+ "mocha": "^7.0.1"
}
}
diff --git a/extensions/css-language-features/schemas/package.schema.json b/extensions/css-language-features/schemas/package.schema.json
index 3aeca1e040a08..cf4193008ec59 100644
--- a/extensions/css-language-features/schemas/package.schema.json
+++ b/extensions/css-language-features/schemas/package.schema.json
@@ -1,5 +1,5 @@
{
- "$schema": "http://json-schema.org/draft-04/schema#",
+ "$schema": "http://json-schema.org/draft-07/schema#",
"title": "CSS contributions to package.json",
"type": "object",
"properties": {
diff --git a/extensions/css-language-features/server/extension-browser.webpack.config.js b/extensions/css-language-features/server/extension-browser.webpack.config.js
new file mode 100644
index 0000000000000..38816259ddf9c
--- /dev/null
+++ b/extensions/css-language-features/server/extension-browser.webpack.config.js
@@ -0,0 +1,23 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+//@ts-check
+
+'use strict';
+
+const withBrowserDefaults = require('../../shared.webpack.config').browser;
+const path = require('path');
+
+module.exports = withBrowserDefaults({
+ context: __dirname,
+ entry: {
+ extension: './src/browser/cssServerMain.ts',
+ },
+ output: {
+ filename: 'cssServerMain.js',
+ path: path.join(__dirname, 'dist', 'browser'),
+ libraryTarget: 'var'
+ }
+});
diff --git a/extensions/css-language-features/server/extension.webpack.config.js b/extensions/css-language-features/server/extension.webpack.config.js
index 68b850b377320..531035f636ca1 100644
--- a/extensions/css-language-features/server/extension.webpack.config.js
+++ b/extensions/css-language-features/server/extension.webpack.config.js
@@ -13,10 +13,10 @@ const path = require('path');
module.exports = withDefaults({
context: path.join(__dirname),
entry: {
- extension: './src/cssServerMain.ts',
+ extension: './src/node/cssServerMain.ts',
},
output: {
filename: 'cssServerMain.js',
- path: path.join(__dirname, 'dist')
+ path: path.join(__dirname, 'dist', 'node'),
}
});
diff --git a/extensions/css-language-features/server/package.json b/extensions/css-language-features/server/package.json
index 6d4265225396c..331af0883a934 100644
--- a/extensions/css-language-features/server/package.json
+++ b/extensions/css-language-features/server/package.json
@@ -7,17 +7,19 @@
"engines": {
"node": "*"
},
- "main": "./out/cssServerMain",
+ "main": "./out/node/cssServerMain",
+ "browser": "./dist/browser/cssServerMain",
"dependencies": {
- "vscode-css-languageservice": "^4.0.3-next.19",
- "vscode-languageserver": "^6.0.0-next.3"
+ "vscode-css-languageservice": "^4.3.3",
+ "vscode-languageserver": "7.0.0-next.3",
+ "vscode-uri": "^2.1.2"
},
"devDependencies": {
- "@types/mocha": "2.2.33",
+ "@types/mocha": "7.0.2",
"@types/node": "^12.11.7",
- "glob": "^7.1.4",
- "mocha": "^6.1.4",
- "mocha-junit-reporter": "^1.23.1",
+ "glob": "^7.1.6",
+ "mocha": "^7.1.2",
+ "mocha-junit-reporter": "^1.23.3",
"mocha-multi-reporters": "^1.1.7"
},
"scripts": {
@@ -27,6 +29,6 @@
"install-service-local": "npm install ../../../../vscode-css-languageservice -f",
"install-server-next": "yarn add vscode-languageserver@next",
"install-server-local": "npm install ../../../../vscode-languageserver-node/server -f",
- "test": "../../../node_modules/.bin/mocha"
+ "test": "node ./test/index.js"
}
}
diff --git a/extensions/css-language-features/server/src/browser/cssServerMain.ts b/extensions/css-language-features/server/src/browser/cssServerMain.ts
new file mode 100644
index 0000000000000..13284fadcd956
--- /dev/null
+++ b/extensions/css-language-features/server/src/browser/cssServerMain.ts
@@ -0,0 +1,16 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import { createConnection, BrowserMessageReader, BrowserMessageWriter } from 'vscode-languageserver/browser';
+import { startServer } from '../cssServer';
+
+declare let self: any;
+
+const messageReader = new BrowserMessageReader(self);
+const messageWriter = new BrowserMessageWriter(self);
+
+const connection = createConnection(messageReader, messageWriter);
+
+startServer(connection, {});
diff --git a/extensions/css-language-features/server/src/cssServer.ts b/extensions/css-language-features/server/src/cssServer.ts
new file mode 100644
index 0000000000000..c2666a46c50ed
--- /dev/null
+++ b/extensions/css-language-features/server/src/cssServer.ts
@@ -0,0 +1,373 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import {
+ Connection, TextDocuments, InitializeParams, InitializeResult, ServerCapabilities, ConfigurationRequest, WorkspaceFolder, TextDocumentSyncKind, NotificationType
+} from 'vscode-languageserver';
+import { URI } from 'vscode-uri';
+import { getCSSLanguageService, getSCSSLanguageService, getLESSLanguageService, LanguageSettings, LanguageService, Stylesheet, TextDocument, Position } from 'vscode-css-languageservice';
+import { getLanguageModelCache } from './languageModelCache';
+import { formatError, runSafeAsync } from './utils/runner';
+import { getDocumentContext } from './utils/documentContext';
+import { fetchDataProviders } from './customData';
+import { RequestService, getRequestService } from './requests';
+
+namespace CustomDataChangedNotification {
+ export const type: NotificationType = new NotificationType('css/customDataChanged');
+}
+
+export interface Settings {
+ css: LanguageSettings;
+ less: LanguageSettings;
+ scss: LanguageSettings;
+}
+
+export interface RuntimeEnvironment {
+ file?: RequestService;
+ http?: RequestService
+}
+
+export function startServer(connection: Connection, runtime: RuntimeEnvironment) {
+
+ // Create a text document manager.
+ const documents = new TextDocuments(TextDocument);
+ // Make the text document manager listen on the connection
+ // for open, change and close text document events
+ documents.listen(connection);
+
+ const stylesheets = getLanguageModelCache(10, 60, document => getLanguageService(document).parseStylesheet(document));
+ documents.onDidClose(e => {
+ stylesheets.onDocumentRemoved(e.document);
+ });
+ connection.onShutdown(() => {
+ stylesheets.dispose();
+ });
+
+ let scopedSettingsSupport = false;
+ let foldingRangeLimit = Number.MAX_VALUE;
+ let workspaceFolders: WorkspaceFolder[];
+
+ let dataProvidersReady: Promise = Promise.resolve();
+
+ const languageServices: { [id: string]: LanguageService } = {};
+
+ const notReady = () => Promise.reject('Not Ready');
+ let requestService: RequestService = { getContent: notReady, stat: notReady, readDirectory: notReady };
+
+ // After the server has started the client sends an initialize request. The server receives
+ // in the passed params the rootPath of the workspace plus the client capabilities.
+ connection.onInitialize((params: InitializeParams): InitializeResult => {
+ workspaceFolders = (params).workspaceFolders;
+ if (!Array.isArray(workspaceFolders)) {
+ workspaceFolders = [];
+ if (params.rootPath) {
+ workspaceFolders.push({ name: '', uri: URI.file(params.rootPath).toString() });
+ }
+ }
+
+ requestService = getRequestService(params.initializationOptions.handledSchemas || ['file'], connection, runtime);
+
+ function getClientCapability(name: string, def: T) {
+ const keys = name.split('.');
+ let c: any = params.capabilities;
+ for (let i = 0; c && i < keys.length; i++) {
+ if (!c.hasOwnProperty(keys[i])) {
+ return def;
+ }
+ c = c[keys[i]];
+ }
+ return c;
+ }
+ const snippetSupport = !!getClientCapability('textDocument.completion.completionItem.snippetSupport', false);
+ scopedSettingsSupport = !!getClientCapability('workspace.configuration', false);
+ foldingRangeLimit = getClientCapability('textDocument.foldingRange.rangeLimit', Number.MAX_VALUE);
+
+ languageServices.css = getCSSLanguageService({ fileSystemProvider: requestService, clientCapabilities: params.capabilities });
+ languageServices.scss = getSCSSLanguageService({ fileSystemProvider: requestService, clientCapabilities: params.capabilities });
+ languageServices.less = getLESSLanguageService({ fileSystemProvider: requestService, clientCapabilities: params.capabilities });
+
+ const capabilities: ServerCapabilities = {
+ textDocumentSync: TextDocumentSyncKind.Incremental,
+ completionProvider: snippetSupport ? { resolveProvider: false, triggerCharacters: ['/', '-'] } : undefined,
+ hoverProvider: true,
+ documentSymbolProvider: true,
+ referencesProvider: true,
+ definitionProvider: true,
+ documentHighlightProvider: true,
+ documentLinkProvider: {
+ resolveProvider: false
+ },
+ codeActionProvider: true,
+ renameProvider: true,
+ colorProvider: {},
+ foldingRangeProvider: true,
+ selectionRangeProvider: true
+ };
+ return { capabilities };
+ });
+
+ function getLanguageService(document: TextDocument) {
+ let service = languageServices[document.languageId];
+ if (!service) {
+ connection.console.log('Document type is ' + document.languageId + ', using css instead.');
+ service = languageServices['css'];
+ }
+ return service;
+ }
+
+ let documentSettings: { [key: string]: Thenable } = {};
+ // remove document settings on close
+ documents.onDidClose(e => {
+ delete documentSettings[e.document.uri];
+ });
+ function getDocumentSettings(textDocument: TextDocument): Thenable {
+ if (scopedSettingsSupport) {
+ let promise = documentSettings[textDocument.uri];
+ if (!promise) {
+ const configRequestParam = { items: [{ scopeUri: textDocument.uri, section: textDocument.languageId }] };
+ promise = connection.sendRequest(ConfigurationRequest.type, configRequestParam).then(s => s[0]);
+ documentSettings[textDocument.uri] = promise;
+ }
+ return promise;
+ }
+ return Promise.resolve(undefined);
+ }
+
+ // The settings have changed. Is send on server activation as well.
+ connection.onDidChangeConfiguration(change => {
+ updateConfiguration(change.settings);
+ });
+
+ function updateConfiguration(settings: Settings) {
+ for (const languageId in languageServices) {
+ languageServices[languageId].configure((settings as any)[languageId]);
+ }
+ // reset all document settings
+ documentSettings = {};
+ // Revalidate any open text documents
+ documents.all().forEach(triggerValidation);
+ }
+
+ const pendingValidationRequests: { [uri: string]: NodeJS.Timer } = {};
+ const validationDelayMs = 500;
+
+ // The content of a text document has changed. This event is emitted
+ // when the text document first opened or when its content has changed.
+ documents.onDidChangeContent(change => {
+ triggerValidation(change.document);
+ });
+
+ // a document has closed: clear all diagnostics
+ documents.onDidClose(event => {
+ cleanPendingValidation(event.document);
+ connection.sendDiagnostics({ uri: event.document.uri, diagnostics: [] });
+ });
+
+ function cleanPendingValidation(textDocument: TextDocument): void {
+ const request = pendingValidationRequests[textDocument.uri];
+ if (request) {
+ clearTimeout(request);
+ delete pendingValidationRequests[textDocument.uri];
+ }
+ }
+
+ function triggerValidation(textDocument: TextDocument): void {
+ cleanPendingValidation(textDocument);
+ pendingValidationRequests[textDocument.uri] = setTimeout(() => {
+ delete pendingValidationRequests[textDocument.uri];
+ validateTextDocument(textDocument);
+ }, validationDelayMs);
+ }
+
+ function validateTextDocument(textDocument: TextDocument): void {
+ const settingsPromise = getDocumentSettings(textDocument);
+ Promise.all([settingsPromise, dataProvidersReady]).then(async ([settings]) => {
+ const stylesheet = stylesheets.get(textDocument);
+ const diagnostics = getLanguageService(textDocument).doValidation(textDocument, stylesheet, settings);
+ // Send the computed diagnostics to VSCode.
+ connection.sendDiagnostics({ uri: textDocument.uri, diagnostics });
+ }, e => {
+ connection.console.error(formatError(`Error while validating ${textDocument.uri}`, e));
+ });
+ }
+
+
+ function updateDataProviders(dataPaths: string[]) {
+ dataProvidersReady = fetchDataProviders(dataPaths, requestService).then(customDataProviders => {
+ for (const lang in languageServices) {
+ languageServices[lang].setDataProviders(true, customDataProviders);
+ }
+ });
+ }
+
+ connection.onCompletion((textDocumentPosition, token) => {
+ return runSafeAsync(async () => {
+ const document = documents.get(textDocumentPosition.textDocument.uri);
+ if (document) {
+ await dataProvidersReady;
+ const styleSheet = stylesheets.get(document);
+ const documentContext = getDocumentContext(document.uri, workspaceFolders);
+ return getLanguageService(document).doComplete2(document, textDocumentPosition.position, styleSheet, documentContext);
+ }
+ return null;
+ }, null, `Error while computing completions for ${textDocumentPosition.textDocument.uri}`, token);
+ });
+
+ connection.onHover((textDocumentPosition, token) => {
+ return runSafeAsync(async () => {
+ const document = documents.get(textDocumentPosition.textDocument.uri);
+ if (document) {
+ await dataProvidersReady;
+ const styleSheet = stylesheets.get(document);
+ return getLanguageService(document).doHover(document, textDocumentPosition.position, styleSheet);
+ }
+ return null;
+ }, null, `Error while computing hover for ${textDocumentPosition.textDocument.uri}`, token);
+ });
+
+ connection.onDocumentSymbol((documentSymbolParams, token) => {
+ return runSafeAsync(async () => {
+ const document = documents.get(documentSymbolParams.textDocument.uri);
+ if (document) {
+ await dataProvidersReady;
+ const stylesheet = stylesheets.get(document);
+ return getLanguageService(document).findDocumentSymbols(document, stylesheet);
+ }
+ return [];
+ }, [], `Error while computing document symbols for ${documentSymbolParams.textDocument.uri}`, token);
+ });
+
+ connection.onDefinition((documentDefinitionParams, token) => {
+ return runSafeAsync(async () => {
+ const document = documents.get(documentDefinitionParams.textDocument.uri);
+ if (document) {
+ await dataProvidersReady;
+ const stylesheet = stylesheets.get(document);
+ return getLanguageService(document).findDefinition(document, documentDefinitionParams.position, stylesheet);
+ }
+ return null;
+ }, null, `Error while computing definitions for ${documentDefinitionParams.textDocument.uri}`, token);
+ });
+
+ connection.onDocumentHighlight((documentHighlightParams, token) => {
+ return runSafeAsync(async () => {
+ const document = documents.get(documentHighlightParams.textDocument.uri);
+ if (document) {
+ await dataProvidersReady;
+ const stylesheet = stylesheets.get(document);
+ return getLanguageService(document).findDocumentHighlights(document, documentHighlightParams.position, stylesheet);
+ }
+ return [];
+ }, [], `Error while computing document highlights for ${documentHighlightParams.textDocument.uri}`, token);
+ });
+
+
+ connection.onDocumentLinks(async (documentLinkParams, token) => {
+ return runSafeAsync(async () => {
+ const document = documents.get(documentLinkParams.textDocument.uri);
+ if (document) {
+ await dataProvidersReady;
+ const documentContext = getDocumentContext(document.uri, workspaceFolders);
+ const stylesheet = stylesheets.get(document);
+ return getLanguageService(document).findDocumentLinks2(document, stylesheet, documentContext);
+ }
+ return [];
+ }, [], `Error while computing document links for ${documentLinkParams.textDocument.uri}`, token);
+ });
+
+
+ connection.onReferences((referenceParams, token) => {
+ return runSafeAsync(async () => {
+ const document = documents.get(referenceParams.textDocument.uri);
+ if (document) {
+ await dataProvidersReady;
+ const stylesheet = stylesheets.get(document);
+ return getLanguageService(document).findReferences(document, referenceParams.position, stylesheet);
+ }
+ return [];
+ }, [], `Error while computing references for ${referenceParams.textDocument.uri}`, token);
+ });
+
+ connection.onCodeAction((codeActionParams, token) => {
+ return runSafeAsync(async () => {
+ const document = documents.get(codeActionParams.textDocument.uri);
+ if (document) {
+ await dataProvidersReady;
+ const stylesheet = stylesheets.get(document);
+ return getLanguageService(document).doCodeActions(document, codeActionParams.range, codeActionParams.context, stylesheet);
+ }
+ return [];
+ }, [], `Error while computing code actions for ${codeActionParams.textDocument.uri}`, token);
+ });
+
+ connection.onDocumentColor((params, token) => {
+ return runSafeAsync(async () => {
+ const document = documents.get(params.textDocument.uri);
+ if (document) {
+ await dataProvidersReady;
+ const stylesheet = stylesheets.get(document);
+ return getLanguageService(document).findDocumentColors(document, stylesheet);
+ }
+ return [];
+ }, [], `Error while computing document colors for ${params.textDocument.uri}`, token);
+ });
+
+ connection.onColorPresentation((params, token) => {
+ return runSafeAsync(async () => {
+ const document = documents.get(params.textDocument.uri);
+ if (document) {
+ await dataProvidersReady;
+ const stylesheet = stylesheets.get(document);
+ return getLanguageService(document).getColorPresentations(document, stylesheet, params.color, params.range);
+ }
+ return [];
+ }, [], `Error while computing color presentations for ${params.textDocument.uri}`, token);
+ });
+
+ connection.onRenameRequest((renameParameters, token) => {
+ return runSafeAsync(async () => {
+ const document = documents.get(renameParameters.textDocument.uri);
+ if (document) {
+ await dataProvidersReady;
+ const stylesheet = stylesheets.get(document);
+ return getLanguageService(document).doRename(document, renameParameters.position, renameParameters.newName, stylesheet);
+ }
+ return null;
+ }, null, `Error while computing renames for ${renameParameters.textDocument.uri}`, token);
+ });
+
+ connection.onFoldingRanges((params, token) => {
+ return runSafeAsync(async () => {
+ const document = documents.get(params.textDocument.uri);
+ if (document) {
+ await dataProvidersReady;
+ return getLanguageService(document).getFoldingRanges(document, { rangeLimit: foldingRangeLimit });
+ }
+ return null;
+ }, null, `Error while computing folding ranges for ${params.textDocument.uri}`, token);
+ });
+
+ connection.onSelectionRanges((params, token) => {
+ return runSafeAsync(async () => {
+ const document = documents.get(params.textDocument.uri);
+ const positions: Position[] = params.positions;
+
+ if (document) {
+ await dataProvidersReady;
+ const stylesheet = stylesheets.get(document);
+ return getLanguageService(document).getSelectionRanges(document, positions, stylesheet);
+ }
+ return [];
+ }, [], `Error while computing selection ranges for ${params.textDocument.uri}`, token);
+ });
+
+ connection.onNotification(CustomDataChangedNotification.type, updateDataProviders);
+
+ // Listen on the connection
+ connection.listen();
+
+}
+
+
diff --git a/extensions/css-language-features/server/src/cssServerMain.ts b/extensions/css-language-features/server/src/cssServerMain.ts
deleted file mode 100644
index 6a9db0b77e779..0000000000000
--- a/extensions/css-language-features/server/src/cssServerMain.ts
+++ /dev/null
@@ -1,390 +0,0 @@
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-
-import {
- createConnection, IConnection, TextDocuments, InitializeParams, InitializeResult, ServerCapabilities, ConfigurationRequest, WorkspaceFolder, TextDocumentSyncKind
-} from 'vscode-languageserver';
-import { URI } from 'vscode-uri';
-import { stat as fsStat } from 'fs';
-import { getCSSLanguageService, getSCSSLanguageService, getLESSLanguageService, LanguageSettings, LanguageService, Stylesheet, FileSystemProvider, FileType, TextDocument, CompletionList, Position } from 'vscode-css-languageservice';
-import { getLanguageModelCache } from './languageModelCache';
-import { getPathCompletionParticipant } from './pathCompletion';
-import { formatError, runSafe, runSafeAsync } from './utils/runner';
-import { getDocumentContext } from './utils/documentContext';
-import { getDataProviders } from './customData';
-
-export interface Settings {
- css: LanguageSettings;
- less: LanguageSettings;
- scss: LanguageSettings;
-}
-
-// Create a connection for the server.
-const connection: IConnection = createConnection();
-
-console.log = connection.console.log.bind(connection.console);
-console.error = connection.console.error.bind(connection.console);
-
-process.on('unhandledRejection', (e: any) => {
- connection.console.error(formatError(`Unhandled exception`, e));
-});
-
-// Create a text document manager.
-const documents = new TextDocuments(TextDocument);
-// Make the text document manager listen on the connection
-// for open, change and close text document events
-documents.listen(connection);
-
-const stylesheets = getLanguageModelCache(10, 60, document => getLanguageService(document).parseStylesheet(document));
-documents.onDidClose(e => {
- stylesheets.onDocumentRemoved(e.document);
-});
-connection.onShutdown(() => {
- stylesheets.dispose();
-});
-
-let scopedSettingsSupport = false;
-let foldingRangeLimit = Number.MAX_VALUE;
-let workspaceFolders: WorkspaceFolder[];
-
-const languageServices: { [id: string]: LanguageService } = {};
-
-const fileSystemProvider: FileSystemProvider = {
- stat(documentUri: string) {
- const filePath = URI.parse(documentUri).fsPath;
-
- return new Promise((c, e) => {
- fsStat(filePath, (err, stats) => {
- if (err) {
- if (err.code === 'ENOENT') {
- return c({
- type: FileType.Unknown,
- ctime: -1,
- mtime: -1,
- size: -1
- });
- } else {
- return e(err);
- }
- }
-
- let type = FileType.Unknown;
- if (stats.isFile()) {
- type = FileType.File;
- } else if (stats.isDirectory()) {
- type = FileType.Directory;
- } else if (stats.isSymbolicLink()) {
- type = FileType.SymbolicLink;
- }
-
- c({
- type,
- ctime: stats.ctime.getTime(),
- mtime: stats.mtime.getTime(),
- size: stats.size
- });
- });
- });
- }
-};
-
-// After the server has started the client sends an initialize request. The server receives
-// in the passed params the rootPath of the workspace plus the client capabilities.
-connection.onInitialize((params: InitializeParams): InitializeResult => {
- workspaceFolders = (params).workspaceFolders;
- if (!Array.isArray(workspaceFolders)) {
- workspaceFolders = [];
- if (params.rootPath) {
- workspaceFolders.push({ name: '', uri: URI.file(params.rootPath).toString() });
- }
- }
-
- const dataPaths: string[] = params.initializationOptions.dataPaths || [];
- const customDataProviders = getDataProviders(dataPaths);
-
- function getClientCapability(name: string, def: T) {
- const keys = name.split('.');
- let c: any = params.capabilities;
- for (let i = 0; c && i < keys.length; i++) {
- if (!c.hasOwnProperty(keys[i])) {
- return def;
- }
- c = c[keys[i]];
- }
- return c;
- }
- const snippetSupport = !!getClientCapability('textDocument.completion.completionItem.snippetSupport', false);
- scopedSettingsSupport = !!getClientCapability('workspace.configuration', false);
- foldingRangeLimit = getClientCapability('textDocument.foldingRange.rangeLimit', Number.MAX_VALUE);
-
- languageServices.css = getCSSLanguageService({ customDataProviders, fileSystemProvider, clientCapabilities: params.capabilities });
- languageServices.scss = getSCSSLanguageService({ customDataProviders, fileSystemProvider, clientCapabilities: params.capabilities });
- languageServices.less = getLESSLanguageService({ customDataProviders, fileSystemProvider, clientCapabilities: params.capabilities });
-
- const capabilities: ServerCapabilities = {
- textDocumentSync: TextDocumentSyncKind.Incremental,
- completionProvider: snippetSupport ? { resolveProvider: false, triggerCharacters: ['/', '-'] } : undefined,
- hoverProvider: true,
- documentSymbolProvider: true,
- referencesProvider: true,
- definitionProvider: true,
- documentHighlightProvider: true,
- documentLinkProvider: {
- resolveProvider: false
- },
- codeActionProvider: true,
- renameProvider: true,
- colorProvider: {},
- foldingRangeProvider: true,
- selectionRangeProvider: true
- };
- return { capabilities };
-});
-
-function getLanguageService(document: TextDocument) {
- let service = languageServices[document.languageId];
- if (!service) {
- connection.console.log('Document type is ' + document.languageId + ', using css instead.');
- service = languageServices['css'];
- }
- return service;
-}
-
-let documentSettings: { [key: string]: Thenable } = {};
-// remove document settings on close
-documents.onDidClose(e => {
- delete documentSettings[e.document.uri];
-});
-function getDocumentSettings(textDocument: TextDocument): Thenable {
- if (scopedSettingsSupport) {
- let promise = documentSettings[textDocument.uri];
- if (!promise) {
- const configRequestParam = { items: [{ scopeUri: textDocument.uri, section: textDocument.languageId }] };
- promise = connection.sendRequest(ConfigurationRequest.type, configRequestParam).then(s => s[0]);
- documentSettings[textDocument.uri] = promise;
- }
- return promise;
- }
- return Promise.resolve(undefined);
-}
-
-// The settings have changed. Is send on server activation as well.
-connection.onDidChangeConfiguration(change => {
- updateConfiguration(change.settings);
-});
-
-function updateConfiguration(settings: Settings) {
- for (const languageId in languageServices) {
- languageServices[languageId].configure((settings as any)[languageId]);
- }
- // reset all document settings
- documentSettings = {};
- // Revalidate any open text documents
- documents.all().forEach(triggerValidation);
-}
-
-const pendingValidationRequests: { [uri: string]: NodeJS.Timer } = {};
-const validationDelayMs = 500;
-
-// The content of a text document has changed. This event is emitted
-// when the text document first opened or when its content has changed.
-documents.onDidChangeContent(change => {
- triggerValidation(change.document);
-});
-
-// a document has closed: clear all diagnostics
-documents.onDidClose(event => {
- cleanPendingValidation(event.document);
- connection.sendDiagnostics({ uri: event.document.uri, diagnostics: [] });
-});
-
-function cleanPendingValidation(textDocument: TextDocument): void {
- const request = pendingValidationRequests[textDocument.uri];
- if (request) {
- clearTimeout(request);
- delete pendingValidationRequests[textDocument.uri];
- }
-}
-
-function triggerValidation(textDocument: TextDocument): void {
- cleanPendingValidation(textDocument);
- pendingValidationRequests[textDocument.uri] = setTimeout(() => {
- delete pendingValidationRequests[textDocument.uri];
- validateTextDocument(textDocument);
- }, validationDelayMs);
-}
-
-function validateTextDocument(textDocument: TextDocument): void {
- const settingsPromise = getDocumentSettings(textDocument);
- settingsPromise.then(settings => {
- const stylesheet = stylesheets.get(textDocument);
- const diagnostics = getLanguageService(textDocument).doValidation(textDocument, stylesheet, settings);
- // Send the computed diagnostics to VSCode.
- connection.sendDiagnostics({ uri: textDocument.uri, diagnostics });
- }, e => {
- connection.console.error(formatError(`Error while validating ${textDocument.uri}`, e));
- });
-}
-
-connection.onCompletion((textDocumentPosition, token) => {
- return runSafe(() => {
- const document = documents.get(textDocumentPosition.textDocument.uri);
- if (!document) {
- return null;
- }
- const cssLS = getLanguageService(document);
- const pathCompletionList: CompletionList = {
- isIncomplete: false,
- items: []
- };
- cssLS.setCompletionParticipants([getPathCompletionParticipant(document, workspaceFolders, pathCompletionList)]);
- const result = cssLS.doComplete(document, textDocumentPosition.position, stylesheets.get(document));
- return {
- isIncomplete: pathCompletionList.isIncomplete,
- items: [...pathCompletionList.items, ...result.items]
- };
- }, null, `Error while computing completions for ${textDocumentPosition.textDocument.uri}`, token);
-});
-
-connection.onHover((textDocumentPosition, token) => {
- return runSafe(() => {
- const document = documents.get(textDocumentPosition.textDocument.uri);
- if (document) {
- const styleSheet = stylesheets.get(document);
- return getLanguageService(document).doHover(document, textDocumentPosition.position, styleSheet);
- }
- return null;
- }, null, `Error while computing hover for ${textDocumentPosition.textDocument.uri}`, token);
-});
-
-connection.onDocumentSymbol((documentSymbolParams, token) => {
- return runSafe(() => {
- const document = documents.get(documentSymbolParams.textDocument.uri);
- if (document) {
- const stylesheet = stylesheets.get(document);
- return getLanguageService(document).findDocumentSymbols(document, stylesheet);
- }
- return [];
- }, [], `Error while computing document symbols for ${documentSymbolParams.textDocument.uri}`, token);
-});
-
-connection.onDefinition((documentDefinitionParams, token) => {
- return runSafe(() => {
- const document = documents.get(documentDefinitionParams.textDocument.uri);
- if (document) {
-
- const stylesheet = stylesheets.get(document);
- return getLanguageService(document).findDefinition(document, documentDefinitionParams.position, stylesheet);
- }
- return null;
- }, null, `Error while computing definitions for ${documentDefinitionParams.textDocument.uri}`, token);
-});
-
-connection.onDocumentHighlight((documentHighlightParams, token) => {
- return runSafe(() => {
- const document = documents.get(documentHighlightParams.textDocument.uri);
- if (document) {
- const stylesheet = stylesheets.get(document);
- return getLanguageService(document).findDocumentHighlights(document, documentHighlightParams.position, stylesheet);
- }
- return [];
- }, [], `Error while computing document highlights for ${documentHighlightParams.textDocument.uri}`, token);
-});
-
-
-connection.onDocumentLinks(async (documentLinkParams, token) => {
- return runSafeAsync(async () => {
- const document = documents.get(documentLinkParams.textDocument.uri);
- if (document) {
- const documentContext = getDocumentContext(document.uri, workspaceFolders);
- const stylesheet = stylesheets.get(document);
- return await getLanguageService(document).findDocumentLinks2(document, stylesheet, documentContext);
- }
- return [];
- }, [], `Error while computing document links for ${documentLinkParams.textDocument.uri}`, token);
-});
-
-
-connection.onReferences((referenceParams, token) => {
- return runSafe(() => {
- const document = documents.get(referenceParams.textDocument.uri);
- if (document) {
- const stylesheet = stylesheets.get(document);
- return getLanguageService(document).findReferences(document, referenceParams.position, stylesheet);
- }
- return [];
- }, [], `Error while computing references for ${referenceParams.textDocument.uri}`, token);
-});
-
-connection.onCodeAction((codeActionParams, token) => {
- return runSafe(() => {
- const document = documents.get(codeActionParams.textDocument.uri);
- if (document) {
- const stylesheet = stylesheets.get(document);
- return getLanguageService(document).doCodeActions(document, codeActionParams.range, codeActionParams.context, stylesheet);
- }
- return [];
- }, [], `Error while computing code actions for ${codeActionParams.textDocument.uri}`, token);
-});
-
-connection.onDocumentColor((params, token) => {
- return runSafe(() => {
- const document = documents.get(params.textDocument.uri);
- if (document) {
- const stylesheet = stylesheets.get(document);
- return getLanguageService(document).findDocumentColors(document, stylesheet);
- }
- return [];
- }, [], `Error while computing document colors for ${params.textDocument.uri}`, token);
-});
-
-connection.onColorPresentation((params, token) => {
- return runSafe(() => {
- const document = documents.get(params.textDocument.uri);
- if (document) {
- const stylesheet = stylesheets.get(document);
- return getLanguageService(document).getColorPresentations(document, stylesheet, params.color, params.range);
- }
- return [];
- }, [], `Error while computing color presentations for ${params.textDocument.uri}`, token);
-});
-
-connection.onRenameRequest((renameParameters, token) => {
- return runSafe(() => {
- const document = documents.get(renameParameters.textDocument.uri);
- if (document) {
- const stylesheet = stylesheets.get(document);
- return getLanguageService(document).doRename(document, renameParameters.position, renameParameters.newName, stylesheet);
- }
- return null;
- }, null, `Error while computing renames for ${renameParameters.textDocument.uri}`, token);
-});
-
-connection.onFoldingRanges((params, token) => {
- return runSafe(() => {
- const document = documents.get(params.textDocument.uri);
- if (document) {
- return getLanguageService(document).getFoldingRanges(document, { rangeLimit: foldingRangeLimit });
- }
- return null;
- }, null, `Error while computing folding ranges for ${params.textDocument.uri}`, token);
-});
-
-connection.onSelectionRanges((params, token) => {
- return runSafe(() => {
- const document = documents.get(params.textDocument.uri);
- const positions: Position[] = params.positions;
-
- if (document) {
- const stylesheet = stylesheets.get(document);
- return getLanguageService(document).getSelectionRanges(document, positions, stylesheet);
- }
- return [];
- }, [], `Error while computing selection ranges for ${params.textDocument.uri}`, token);
-});
-
-
-// Listen on the connection
-connection.listen();
diff --git a/extensions/css-language-features/server/src/customData.ts b/extensions/css-language-features/server/src/customData.ts
index f173d884a2b24..ccfc706452c27 100644
--- a/extensions/css-language-features/server/src/customData.ts
+++ b/extensions/css-language-features/server/src/customData.ts
@@ -3,48 +3,36 @@
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
-import { CSSDataV1, ICSSDataProvider } from 'vscode-css-languageservice';
-import * as fs from 'fs';
+import { ICSSDataProvider, newCSSDataProvider } from 'vscode-css-languageservice';
+import { RequestService } from './requests';
-export function getDataProviders(dataPaths: string[]): ICSSDataProvider[] {
- const providers = dataPaths.map(p => {
- if (fs.existsSync(p)) {
- const data = parseCSSData(fs.readFileSync(p, 'utf-8'));
- return {
- provideProperties: () => data.properties || [],
- provideAtDirectives: () => data.atDirectives || [],
- providePseudoClasses: () => data.pseudoClasses || [],
- providePseudoElements: () => data.pseudoElements || []
- };
- } else {
- return {
- provideProperties: () => [],
- provideAtDirectives: () => [],
- providePseudoClasses: () => [],
- providePseudoElements: () => []
- };
+export function fetchDataProviders(dataPaths: string[], requestService: RequestService): Promise {
+ const providers = dataPaths.map(async p => {
+ try {
+ const content = await requestService.getContent(p);
+ return parseCSSData(content);
+ } catch (e) {
+ return newCSSDataProvider({ version: 1 });
}
});
- return providers;
+ return Promise.all(providers);
}
-function parseCSSData(source: string): CSSDataV1 {
+function parseCSSData(source: string): ICSSDataProvider {
let rawData: any;
try {
rawData = JSON.parse(source);
} catch (err) {
- return {
- version: 1
- };
+ return newCSSDataProvider({ version: 1 });
}
- return {
- version: 1,
+ return newCSSDataProvider({
+ version: rawData.version || 1,
properties: rawData.properties || [],
atDirectives: rawData.atDirectives || [],
pseudoClasses: rawData.pseudoClasses || [],
pseudoElements: rawData.pseudoElements || []
- };
+ });
}
diff --git a/extensions/css-language-features/server/src/node/cssServerMain.ts b/extensions/css-language-features/server/src/node/cssServerMain.ts
new file mode 100644
index 0000000000000..9e145398ff15b
--- /dev/null
+++ b/extensions/css-language-features/server/src/node/cssServerMain.ts
@@ -0,0 +1,21 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import { createConnection, Connection } from 'vscode-languageserver/node';
+import { formatError } from '../utils/runner';
+import { startServer } from '../cssServer';
+import { getNodeFSRequestService } from './nodeFs';
+
+// Create a connection for the server.
+const connection: Connection = createConnection();
+
+console.log = connection.console.log.bind(connection.console);
+console.error = connection.console.error.bind(connection.console);
+
+process.on('unhandledRejection', (e: any) => {
+ connection.console.error(formatError(`Unhandled exception`, e));
+});
+
+startServer(connection, { file: getNodeFSRequestService() });
diff --git a/extensions/css-language-features/server/src/node/nodeFs.ts b/extensions/css-language-features/server/src/node/nodeFs.ts
new file mode 100644
index 0000000000000..c7b1301296d4a
--- /dev/null
+++ b/extensions/css-language-features/server/src/node/nodeFs.ts
@@ -0,0 +1,87 @@
+/*---------------------------------------------------------------------------------------------
+ * Copyright (c) Microsoft Corporation. All rights reserved.
+ * Licensed under the MIT License. See License.txt in the project root for license information.
+ *--------------------------------------------------------------------------------------------*/
+
+import { RequestService, getScheme } from '../requests';
+import { URI as Uri } from 'vscode-uri';
+
+import * as fs from 'fs';
+import { FileType } from 'vscode-css-languageservice';
+
+export function getNodeFSRequestService(): RequestService {
+ function ensureFileUri(location: string) {
+ if (getScheme(location) !== 'file') {
+ throw new Error('fileRequestService can only handle file URLs');
+ }
+ }
+ return {
+ getContent(location: string, encoding?: string) {
+ ensureFileUri(location);
+ return new Promise((c, e) => {
+ const uri = Uri.parse(location);
+ fs.readFile(uri.fsPath, encoding, (err, buf) => {
+ if (err) {
+ return e(err);
+ }
+ c(buf.toString());
+
+ });
+ });
+ },
+ stat(location: string) {
+ ensureFileUri(location);
+ return new Promise((c, e) => {
+ const uri = Uri.parse(location);
+ fs.stat(uri.fsPath, (err, stats) => {
+ if (err) {
+ if (err.code === 'ENOENT') {
+ return c({ type: FileType.Unknown, ctime: -1, mtime: -1, size: -1 });
+ } else {
+ return e(err);
+ }
+ }
+
+ let type = FileType.Unknown;
+ if (stats.isFile()) {
+ type = FileType.File;
+ } else if (stats.isDirectory()) {
+ type = FileType.Directory;
+ } else if (stats.isSymbolicLink()) {
+ type = FileType.SymbolicLink;
+ }
+
+ c({
+ type,
+ ctime: stats.ctime.getTime(),
+ mtime: stats.mtime.getTime(),
+ size: stats.size
+ });
+ });
+ });
+ },
+ readDirectory(location: string) {
+ ensureFileUri(location);
+ return new Promise((c, e) => {
+ const path = Uri.parse(location).fsPath;
+
+ fs.readdir(path, { withFileTypes: true }, (err, children) => {
+ if (err) {
+ return e(err);
+ }
+ c(children.map(stat => {
+ if (stat.isSymbolicLink()) {
+ return [stat.name, FileType.SymbolicLink];
+ } else if (stat.isDirectory()) {
+ return [stat.name, FileType.Directory];
+ } else if (stat.isFile()) {
+ return [stat.name, FileType.File];
+ } else {
+ return [stat.name, FileType.Unknown];
+ }
+ }));
+ });
+ });
+ }
+ };
+}
diff --git a/extensions/css-language-features/server/src/pathCompletion.ts b/extensions/css-language-features/server/src/pathCompletion.ts
deleted file mode 100644
index 6862f28e03489..0000000000000
--- a/extensions/css-language-features/server/src/pathCompletion.ts
+++ /dev/null
@@ -1,213 +0,0 @@
-/*---------------------------------------------------------------------------------------------
- * Copyright (c) Microsoft Corporation. All rights reserved.
- * Licensed under the MIT License. See License.txt in the project root for license information.
- *--------------------------------------------------------------------------------------------*/
-
-import * as path from 'path';
-import * as fs from 'fs';
-import { URI } from 'vscode-uri';
-
-import { TextDocument, CompletionList, CompletionItemKind, CompletionItem, TextEdit, Range, Position } from 'vscode-languageserver-types';
-import { WorkspaceFolder } from 'vscode-languageserver';
-import { ICompletionParticipant } from 'vscode-css-languageservice';
-
-import { startsWith, endsWith } from './utils/strings';
-
-export function getPathCompletionParticipant(
- document: TextDocument,
- workspaceFolders: WorkspaceFolder[],
- result: CompletionList
-): ICompletionParticipant {
- return {
- onCssURILiteralValue: ({ position, range, uriValue }) => {
- const fullValue = stripQuotes(uriValue);
- if (!shouldDoPathCompletion(uriValue, workspaceFolders)) {
- if (fullValue === '.' || fullValue === '..') {
- result.isIncomplete = true;
- }
- return;
- }
-
- let suggestions = providePathSuggestions(uriValue, position, range, document, workspaceFolders);
- result.items = [...suggestions, ...result.items];
- },
- onCssImportPath: ({ position, range, pathValue }) => {
- const fullValue = stripQuotes(pathValue);
- if (!shouldDoPathCompletion(pathValue, workspaceFolders)) {
- if (fullValue === '.' || fullValue === '..') {
- result.isIncomplete = true;
- }
- return;
- }
-
- let suggestions = providePathSuggestions(pathValue, position, range, document, workspaceFolders);
-
- if (document.languageId === 'scss') {
- suggestions.forEach(s => {
- if (startsWith(s.label, '_') && endsWith(s.label, '.scss')) {
- if (s.textEdit) {
- s.textEdit.newText = s.label.slice(1, -5);
- } else {
- s.label = s.label.slice(1, -5);
- }
- }
- });
- }
-
- result.items = [...suggestions, ...result.items];
- }
- };
-}
-
-function providePathSuggestions(pathValue: string, position: Position, range: Range, document: TextDocument, workspaceFolders: WorkspaceFolder[]) {
- const fullValue = stripQuotes(pathValue);
- const isValueQuoted = startsWith(pathValue, `'`) || startsWith(pathValue, `"`);
- const valueBeforeCursor = isValueQuoted
- ? fullValue.slice(0, position.character - (range.start.character + 1))
- : fullValue.slice(0, position.character - range.start.character);
- const workspaceRoot = resolveWorkspaceRoot(document, workspaceFolders);
- const currentDocFsPath = URI.parse(document.uri).fsPath;
-
- const paths = providePaths(valueBeforeCursor, currentDocFsPath, workspaceRoot)
- .filter(p => {
- // Exclude current doc's path
- return path.resolve(currentDocFsPath, '../', p) !== currentDocFsPath;
- })
- .filter(p => {
- // Exclude paths that start with `.`
- return p[0] !== '.';
- });
-
- const fullValueRange = isValueQuoted ? shiftRange(range, 1, -1) : range;
- const replaceRange = pathToReplaceRange(valueBeforeCursor, fullValue, fullValueRange);
-
- const suggestions = paths.map(p => pathToSuggestion(p, replaceRange));
- return suggestions;
-}
-
-function shouldDoPathCompletion(pathValue: string, workspaceFolders: WorkspaceFolder[]): boolean {
- const fullValue = stripQuotes(pathValue);
- if (fullValue === '.' || fullValue === '..') {
- return false;
- }
-
- if (!workspaceFolders || workspaceFolders.length === 0) {
- return false;
- }
-
- return true;
-}
-
-function stripQuotes(fullValue: string) {
- if (startsWith(fullValue, `'`) || startsWith(fullValue, `"`)) {
- return fullValue.slice(1, -1);
- } else {
- return fullValue;
- }
-}
-
-/**
- * Get a list of path suggestions. Folder suggestions are suffixed with a slash.
- */
-function providePaths(valueBeforeCursor: string, activeDocFsPath: string, root?: string): string[] {
- const lastIndexOfSlash = valueBeforeCursor.lastIndexOf('/');
- const valueBeforeLastSlash = valueBeforeCursor.slice(0, lastIndexOfSlash + 1);
-
- const startsWithSlash = startsWith(valueBeforeCursor, '/');
- let parentDir: string;
- if (startsWithSlash) {
- if (!root) {
- return [];
- }
- parentDir = path.resolve(root, '.' + valueBeforeLastSlash);
- } else {
- parentDir = path.resolve(activeDocFsPath, '..', valueBeforeLastSlash);
- }
-
- try {
- return fs.readdirSync(parentDir).map(f => {
- return isDir(path.resolve(parentDir, f))
- ? f + '/'
- : f;
- });
- } catch (e) {
- return [];
- }
-}
-
-const isDir = (p: string) => {
- try {
- return fs.statSync(p).isDirectory();
- } catch (e) {
- return false;
- }
-};
-
-function pathToReplaceRange(valueBeforeCursor: string, fullValue: string, fullValueRange: Range) {
- let replaceRange: Range;
- const lastIndexOfSlash = valueBeforeCursor.lastIndexOf('/');
- if (lastIndexOfSlash === -1) {
- replaceRange = fullValueRange;
- } else {
- // For cases where cursor is in the middle of attribute value, like ',
+ '',
+ '',
+ '\t$0',
+ '',
+ '