diff --git a/.automation/build.py b/.automation/build.py
index 4ba983e8c8b..8e08a5d5639 100644
--- a/.automation/build.py
+++ b/.automation/build.py
@@ -42,6 +42,7 @@
from webpreview import web_preview
RELEASE = "--release" in sys.argv
+UPDATE_STATS = "--stats" in sys.argv or RELEASE is True
UPDATE_DOC = "--doc" in sys.argv or RELEASE is True
UPDATE_DEPENDENTS = "--dependents" in sys.argv
UPDATE_CHANGELOG = "--changelog" in sys.argv
@@ -89,6 +90,7 @@
HELPS_FILE = REPO_HOME + "/.automation/generated/linter-helps.json"
LINKS_PREVIEW_FILE = REPO_HOME + "/.automation/generated/linter-links-previews.json"
DOCKER_STATS_FILE = REPO_HOME + "/.automation/generated/flavors-stats.json"
+PLUGINS_FILE = REPO_HOME + "/.automation/plugins.yml"
FLAVORS_DIR = REPO_HOME + "/flavors"
LINTERS_DIR = REPO_HOME + "/linters"
GLOBAL_FLAVORS_FILE = REPO_HOME + "/megalinter/descriptors/all_flavors.json"
@@ -104,7 +106,7 @@
IDE_LIST = {
"atom": {"label": "Atom", "url": "https://atom.io/"},
- "brackets": {"label": "Brackets", "url": "http://brackets.io/"},
+ "brackets": {"label": "Brackets", "url": "https://brackets.io/"},
"eclipse": {"label": "Eclipse", "url": "https://www.eclipse.org/"},
"emacs": {"label": "Emacs", "url": "https://www.gnu.org/software/emacs/"},
"idea": {
@@ -116,6 +118,18 @@
"vscode": {"label": "Visual Studio Code", "url": "https://code.visualstudio.com/"},
}
+DEPRECATED_LINTERS = [
+ "CREDENTIALS_SECRETLINT", # Removed in v6
+ "DOCKERFILE_DOCKERFILELINT", # Removed in v6
+ "GIT_GIT_DIFF", # Removed in v6
+ "PHP_BUILTIN", # Removed in v6
+ "KUBERNETES_KUBEVAL", # Removed in v7
+ "REPOSITORY_GOODCHECK", # Removed in v7
+ "SPELL_MISSPELL", # Removed in v7
+ "TERRAFORM_CHECKOV", # Removed in v7
+ "TERRAFORM_KICS", # Removed in v7
+]
+
DESCRIPTORS_FOR_BUILD_CACHE = None
@@ -126,7 +140,7 @@ def generate_all_flavors():
for flavor, flavor_info in flavors.items():
generate_flavor(flavor, flavor_info)
update_mkdocs_and_workflow_yml_with_flavors()
- if UPDATE_DOC is True:
+ if UPDATE_STATS is True:
try:
update_docker_pulls_counter()
except requests.exceptions.ConnectionError as e:
@@ -434,7 +448,7 @@ def build_dockerfile(
apk_install_command = ""
if len(apk_packages) > 0:
apk_install_command = (
- "RUN apk add --update --no-cache \\\n "
+ "RUN apk add --no-cache \\\n "
+ " \\\n ".join(list(dict.fromkeys(apk_packages)))
+ " \\\n && git config --global core.autocrlf true"
)
@@ -788,8 +802,10 @@ def generate_documentation():
+ f"[**{len(linters_by_type['tooling_format'])}** tooling formats](#tooling-formats) "
+ "and **ready to use out of the box**, as a GitHub action or any CI system "
+ "**highly configurable** and **free for all uses**.\n\n"
- + "[**Upgrade to MegaLinter v7 !**]"
- + "https://github.com/oxsecurity/megalinter/issues/2608" # TODOV7: Replace link
+ + "[**Switch to MegaLinter v7 !**]"
+ + "(https://github.com/oxsecurity/megalinter/issues/2692)\n\n"
+ + "[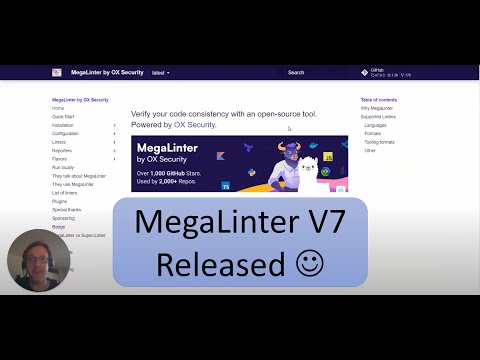]"
+ + "(https://www.youtube.com/watch?v=6NSBzq01S9g)"
)
# Update README.md file
replace_in_file(
@@ -815,6 +831,18 @@ def generate_documentation():
"",
flavors_table_md_str,
)
+
+ # Build & Update flavors table
+ plugins_table_md = build_plugins_md_table()
+ plugins_table_md_str = "\n".join(plugins_table_md)
+ logging.info("Generated Plugins table for README:\n" + plugins_table_md_str)
+ replace_in_file(
+ f"{REPO_HOME}/README.md",
+ "",
+ "",
+ plugins_table_md_str,
+ )
+
# Generate flavors individual documentations
flavors = megalinter.flavor_factory.get_all_flavors()
for flavor, flavor_info in flavors.items():
@@ -1121,7 +1149,9 @@ def process_type(linters_by_type, type1, type_label, linters_tables_md):
linter_doc_md += [f"# {linter.linter_name}\n{md_individual_extra}"]
# Indicate that a linter is disabled in this version
+ title_prefix = ""
if hasattr(linter, "deprecated") and linter.deprecated is True:
+ title_prefix = "(deprecated) "
linter_doc_md += [""]
linter_doc_md += ["> This linter has been deprecated.", ">"]
@@ -1300,7 +1330,7 @@ def process_type(linters_by_type, type1, type_label, linters_tables_md):
{
"$id": f"#/properties/{linter.name}_FILTER_REGEX_INCLUDE",
"type": "string",
- "title": f"{linter.name}: Including Regex",
+ "title": f"{title_prefix}{linter.name}: Including Regex",
},
],
[
@@ -1308,7 +1338,7 @@ def process_type(linters_by_type, type1, type_label, linters_tables_md):
{
"$id": f"#/properties/{linter.name}_FILTER_REGEX_EXCLUDE",
"type": "string",
- "title": f"{linter.name}: Excluding Regex",
+ "title": f"{title_prefix}{linter.name}: Excluding Regex",
},
],
]
@@ -1348,7 +1378,7 @@ def process_type(linters_by_type, type1, type_label, linters_tables_md):
{
"$id": f"#/properties/{linter.name}_CLI_LINT_MODE",
"type": "string",
- "title": f"{linter.name}: Override default cli lint mode",
+ "title": f"{title_prefix}{linter.name}: Override default cli lint mode",
"default": linter.cli_lint_mode,
"enum": enum,
},
@@ -1381,7 +1411,10 @@ def process_type(linters_by_type, type1, type_label, linters_tables_md):
{
"$id": f"#/properties/{linter.name}_FILE_EXTENSIONS",
"type": "array",
- "title": f"{linter.name}: Override descriptor/linter matching files extensions",
+ "title": (
+ title_prefix
+ + f"{linter.name}: Override descriptor/linter matching files extensions"
+ ),
"examples:": [".py", ".myext"],
"items": {"type": "string"},
},
@@ -1391,7 +1424,10 @@ def process_type(linters_by_type, type1, type_label, linters_tables_md):
{
"$id": f"#/properties/{linter.name}_FILE_NAMES_REGEX",
"type": "array",
- "title": f"{linter.name}: Override descriptor/linter matching file name regex",
+ "title": (
+ title_prefix
+ + f"{linter.name}: Override descriptor/linter matching file name regex"
+ ),
"examples": ["Dockerfile(-.+)?", "Jenkinsfile"],
"items": {"type": "string"},
},
@@ -1402,12 +1438,15 @@ def process_type(linters_by_type, type1, type_label, linters_tables_md):
remove_in_config_schema_file(
[f"{linter.name}_FILE_EXTENSIONS", f"{linter.name}_FILE_NAMES_REGEX"]
)
- # Pre/post commands
+ # Pre/post commands & unsecured variables
linter_doc_md += [
f"| {linter.name}_PRE_COMMANDS | List of bash commands to run before the linter"
f"| {dump_as_json(linter.pre_commands,'None')} |",
f"| {linter.name}_POST_COMMANDS | List of bash commands to run after the linter"
f"| {dump_as_json(linter.post_commands,'None')} |",
+ f"| {linter.name}_UNSECURED_ENV_VARIABLES | List of env variables explicitly "
+ + f"not filtered before calling {linter.name} and its pre/post commands"
+ f"| {dump_as_json(linter.post_commands,'None')} |",
]
add_in_config_schema_file(
[
@@ -1416,7 +1455,7 @@ def process_type(linters_by_type, type1, type_label, linters_tables_md):
{
"$id": f"#/properties/{linter.name}_ARGUMENTS",
"type": ["array", "string"],
- "title": f"{linter.name}: Custom arguments",
+ "title": f"{title_prefix}{linter.name}: Custom arguments",
"description": f"{linter.name}: User custom arguments to add in linter CLI call",
"examples:": ["--foo", "bar"],
"items": {"type": "string"},
@@ -1427,7 +1466,10 @@ def process_type(linters_by_type, type1, type_label, linters_tables_md):
{
"$id": f"#/properties/{linter.name}_PRE_COMMANDS",
"type": "array",
- "title": f"{linter.name}: Define or override a list of bash commands to run before the linter",
+ "title": (
+ title_prefix
+ + f"{linter.name}: Define or override a list of bash commands to run before the linter"
+ ),
"examples": [
[
{
@@ -1445,7 +1487,10 @@ def process_type(linters_by_type, type1, type_label, linters_tables_md):
{
"$id": f"#/properties/{linter.name}_POST_COMMANDS",
"type": "array",
- "title": f"{linter.name}: Define or override a list of bash commands to run after the linter",
+ "title": (
+ title_prefix
+ + f"{linter.name}: Define or override a list of bash commands to run after the linter"
+ ),
"examples": [
[
{
@@ -1464,7 +1509,10 @@ def process_type(linters_by_type, type1, type_label, linters_tables_md):
"$id": f"#/properties/{linter.name}_DISABLE_ERRORS",
"type": "boolean",
"default": False,
- "title": f"{linter.name}: Linter doesn't make MegaLinter fail even if errors are found",
+ "title": (
+ title_prefix
+ + f"{linter.name}: Linter doesn't make MegaLinter fail even if errors are found"
+ ),
},
],
[
@@ -1473,7 +1521,7 @@ def process_type(linters_by_type, type1, type_label, linters_tables_md):
"$id": f"#/properties/{linter.name}_DISABLE_ERRORS_IF_LESS_THAN",
"type": "number",
"default": 0,
- "title": f"{linter.name}: Maximum number of errors allowed",
+ "title": f"{title_prefix}{linter.name}: Maximum number of errors allowed",
},
],
[
@@ -1482,7 +1530,19 @@ def process_type(linters_by_type, type1, type_label, linters_tables_md):
"$id": f"#/properties/{linter.name}_CLI_EXECUTABLE",
"type": "array",
"default": [linter.cli_executable],
- "title": f"{linter.name}: CLI Executable",
+ "title": f"{title_prefix}{linter.name}: CLI Executable",
+ "items": {"type": "string"},
+ },
+ ],
+ [
+ f"{linter.name}_UNSECURED_ENV_VARIABLES",
+ {
+ "$id": f"#/properties/{linter.name}_UNSECURED_ENV_VARIABLES",
+ "type": "array",
+ "default": [],
+ "description": "List of env variables explicitly "
+ + f"not filtered before calling {linter.name} and its pre/post commands",
+ "title": f"{title_prefix}{linter.name}: Unsecured env variables",
"items": {"type": "string"},
},
],
@@ -1504,7 +1564,7 @@ def process_type(linters_by_type, type1, type_label, linters_tables_md):
{
"$id": f"#/properties/{linter.name}_CONFIG_FILE",
"type": "string",
- "title": f"{linter.name}: Custom config file name",
+ "title": f"{title_prefix}{linter.name}: Custom config file name",
"default": linter.config_file_name,
"description": f"{linter.name}: User custom config file name if different from default",
},
@@ -1514,7 +1574,7 @@ def process_type(linters_by_type, type1, type_label, linters_tables_md):
{
"$id": f"#/properties/{linter.name}_RULES_PATH",
"type": "string",
- "title": f"{linter.name}: Custom config file path",
+ "title": f"{title_prefix}{linter.name}: Custom config file path",
"description": f"{linter.name}: Path where to find linter configuration file",
},
],
@@ -1542,7 +1602,7 @@ def process_type(linters_by_type, type1, type_label, linters_tables_md):
{
"$id": f"#/properties/{linter.name}_DIRECTORY",
"type": "string",
- "title": f"{linter.name}: Directory containing {linter.descriptor_id} files",
+ "title": f"{title_prefix}{linter.name}: Directory containing {linter.descriptor_id} files",
"default": linter.files_sub_directory,
},
],
@@ -1777,6 +1837,24 @@ def build_flavors_md_table(filter_linter_name=None, replace_link=False):
return md_table
+# Build plugins table from YML file in .automation/plugins.yml
+def build_plugins_md_table():
+ with open(PLUGINS_FILE, "r", encoding="utf-8") as f:
+ plugins_file_data = yaml.safe_load(f)
+ plugins = plugins_file_data["plugins"]
+ md_table = [
+ "| Name | Description | Author | Raw URL |",
+ "| :----- | :---------- | :--------------: | :--- |",
+ ]
+ for plugin in plugins:
+ md_table += [
+ f"| [**{plugin['name']}**]({plugin['docUrl']}) | "
+ f"{plugin['description']} | {plugin['author']} | "
+ f"[Descriptor]({plugin['pluginUrl']}) |",
+ ]
+ return md_table
+
+
def update_mkdocs_and_workflow_yml_with_flavors():
mkdocs_yml = []
gha_workflow_yml = [" flavor:", " ["]
@@ -2220,14 +2298,22 @@ def move_to_file(file_path, start, end, target_file, keep_in_source=False):
with open(file_path, "w", encoding="utf-8") as file:
file.write(file_content)
logging.info("Updated " + file.name + " between " + start + " and " + end)
- bracket_content = (
- bracket_content.replace("####", "#THREE#")
- .replace("###", "#TWO#")
- .replace("##", "#ONE#")
- .replace("#THREE#", "###")
- .replace("#TWO#", "##")
- .replace("#ONE#", "#")
- )
+ if ""
@@ -2259,7 +2345,14 @@ def replace_anchors_by_links(file_path, moves):
["formats", "supported-linters.md#formats"],
["tooling-formats", "supported-linters.md#tooling-formats"],
["other", "supported-linters.md#other"],
- ["apply-fixes", "configuration.md#apply-fixes"],
+ ["apply-fixes", "config-apply-fixes.md"],
+ ["installation", "install-assisted.md"],
+ ["configuration", "config-file.md"],
+ ["activation-and-deactivation", "config-activation.md"],
+ ["filter-linted-files", "config-filtering.md"],
+ ["pre-commands", "config-precommands.md"],
+ ["post-commands", "config-postcommands.md"],
+ ["environment-variables-security", "config-variables-security.md"],
]:
file_content_new = file_content_new.replace(f"(#{pair[0]})", f"({pair[1]})")
if file_content_new != file_content:
@@ -2325,8 +2418,27 @@ def finalize_doc_build():
# 'format',
# 'tooling-formats',
# 'other',
- "installation",
- "configuration",
+ "install-assisted",
+ "install-version",
+ "install-github",
+ "install-gitlab",
+ "install-azure",
+ "install-bitbucket",
+ "install-jenkins",
+ "install-concourse",
+ "install-drone",
+ "install-docker",
+ "install-locally",
+ "config-file",
+ "config-variables",
+ "config-activation",
+ "config-filtering",
+ "config-apply-fixes",
+ "config-linters",
+ "config-precommands",
+ "config-postcommands",
+ "config-variables-security",
+ "config-cli-lint-mode",
"reporters",
"flavors",
"badge",
@@ -2367,7 +2479,7 @@ def finalize_doc_build():
"",
"",
"""
-[](https://megalinter.io/flavors/)
+[](https://megalinter.io/flavors/)
[](https://npmjs.org/package/mega-linter-runner)
[](https://github.com/oxsecurity/megalinter/stargazers/)
[](https://github.com/oxsecurity/megalinter/network/dependents)
@@ -2388,6 +2500,18 @@ def finalize_doc_build():
"",
"",
)
+ replace_in_file(
+ target_file,
+ "",
+ "",
+ "",
+ )
+ replace_in_file(
+ target_file,
+ "",
+ "",
+ "",
+ )
# Remove link to online doc
replace_in_file(
target_file,
@@ -2491,16 +2615,7 @@ def generate_json_schema_enums():
json_schema["definitions"]["enum_descriptor_keys"]["enum"] += ["CREDENTIALS", "GIT"]
json_schema["definitions"]["enum_linter_keys"]["enum"] = [x.name for x in linters]
# Deprecated linters
- json_schema["definitions"]["enum_linter_keys"]["enum"] += [
- "CREDENTIALS_SECRETLINT", # Removed in v6
- "DOCKERFILE_DOCKERFILELINT", # Removed in v6
- "GIT_GIT_DIFF", # Removed in v6
- "PHP_BUILTIN", # Removed in v6
- "KUBERNETES_KUBEVAL", # Removed in v7
- "REPOSITORY_GOODCHECK", # Removed in v7
- "SPELL_MISSPELL", # Removed in v7
- "TERRAFORM_CHECKOV", # Removed in v7
- ]
+ json_schema["definitions"]["enum_linter_keys"]["enum"] += DEPRECATED_LINTERS
with open(CONFIG_JSON_SCHEMA, "w", encoding="utf-8") as outfile:
json.dump(json_schema, outfile, indent=2, sort_keys=True)
outfile.write("\n")
@@ -2668,7 +2783,11 @@ def generate_documentation_all_linters():
# Update license key for licenses file
resp = r.json()
if resp is not None and not isinstance(resp, type(None)):
- if "license" in resp and "spdx_id" in resp["license"]:
+ if (
+ "license" in resp
+ and resp["license"] is not None
+ and "spdx_id" in resp["license"]
+ ):
license = (
resp["license"]["spdx_id"]
if resp["license"]["spdx_id"] != "NOASSERTION"
@@ -2693,12 +2812,22 @@ def generate_documentation_all_linters():
resp_license = r_license.json()
if "download_url" in resp_license:
license_downloaded = session.get(
- resp_license["download_url"]
+ resp_license["download_url"],
+ headers=api_github_headers,
)
with open(
linter_license_md_file, "w", encoding="utf-8"
) as license_out_file:
- license_out_file.write(license_downloaded.text)
+ license_header = (
+ "---\n"
+ f"title: License info for {linter.linter_name} within MegaLinter\n"
+ "search:\n"
+ " exclude: true\n"
+ "---\n"
+ )
+ license_out_file.write(
+ license_header + license_downloaded.text
+ )
logging.info(
f"Copied license of {linter.linter_name} in {linter_license_md_file}"
)
diff --git a/.automation/generated/flavors-stats.json b/.automation/generated/flavors-stats.json
index 1880d0031e3..76d47007a92 100644
--- a/.automation/generated/flavors-stats.json
+++ b/.automation/generated/flavors-stats.json
@@ -1481,8 +1481,164 @@
2948676
],
[
- "2023-05-14T16:41:46",
- 2949395
+ "2023-05-14T19:54:23",
+ 2949683
+ ],
+ [
+ "2023-05-17T23:06:02",
+ 2956012
+ ],
+ [
+ "2023-05-18T18:05:13",
+ 2957431
+ ],
+ [
+ "2023-05-19T18:27:46",
+ 2959002
+ ],
+ [
+ "2023-05-20T06:58:27",
+ 2959618
+ ],
+ [
+ "2023-05-21T17:54:05",
+ 2960551
+ ],
+ [
+ "2023-05-22T19:05:24",
+ 2962658
+ ],
+ [
+ "2023-05-23T01:07:57",
+ 2963164
+ ],
+ [
+ "2023-05-24T01:14:32",
+ 2965365
+ ],
+ [
+ "2023-05-26T01:11:00",
+ 2969496
+ ],
+ [
+ "2023-05-27T17:04:12",
+ 2971966
+ ],
+ [
+ "2023-05-28T17:03:24",
+ 2972895
+ ],
+ [
+ "2023-05-29T11:13:08",
+ 2973698
+ ],
+ [
+ "2023-05-31T23:48:39",
+ 2979928
+ ],
+ [
+ "2023-06-01T01:25:18",
+ 2980280
+ ],
+ [
+ "2023-06-02T01:10:33",
+ 2982873
+ ],
+ [
+ "2023-06-03T09:59:50",
+ 2985399
+ ],
+ [
+ "2023-06-04T01:13:43",
+ 2985820
+ ],
+ [
+ "2023-06-05T01:08:28",
+ 2986683
+ ],
+ [
+ "2023-06-07T01:10:00",
+ 2990975
+ ],
+ [
+ "2023-06-08T05:19:18",
+ 2993233
+ ],
+ [
+ "2023-06-11T18:48:09",
+ 2997453
+ ],
+ [
+ "2023-06-12T01:16:15",
+ 2997733
+ ],
+ [
+ "2023-06-13T01:11:33",
+ 2999733
+ ],
+ [
+ "2023-06-17T01:09:24",
+ 3008097
+ ],
+ [
+ "2023-06-21T05:26:13",
+ 3013604
+ ],
+ [
+ "2023-06-23T01:12:21",
+ 3017176
+ ],
+ [
+ "2023-06-24T01:13:03",
+ 3018770
+ ],
+ [
+ "2023-06-25T01:19:08",
+ 3019324
+ ],
+ [
+ "2023-07-02T01:17:57",
+ 3030041
+ ],
+ [
+ "2023-07-05T04:19:03",
+ 3034027
+ ],
+ [
+ "2023-07-06T01:13:59",
+ 3035756
+ ],
+ [
+ "2023-07-07T01:22:59",
+ 3037762
+ ],
+ [
+ "2023-07-09T01:17:28",
+ 3039856
+ ],
+ [
+ "2023-07-10T12:35:57",
+ 3041527
+ ],
+ [
+ "2023-07-11T18:04:48",
+ 3044556
+ ],
+ [
+ "2023-07-13T01:16:23",
+ 3047030
+ ],
+ [
+ "2023-07-14T18:55:34",
+ 3050109
+ ],
+ [
+ "2023-07-15T14:49:24",
+ 3050879
+ ],
+ [
+ "2023-07-17T01:18:56",
+ 3051957
]
],
"ci_light": [
@@ -2967,8 +3123,164 @@
63857
],
[
- "2023-05-14T16:41:46",
- 63926
+ "2023-05-14T19:54:23",
+ 63946
+ ],
+ [
+ "2023-05-17T23:06:02",
+ 64761
+ ],
+ [
+ "2023-05-18T18:05:13",
+ 64933
+ ],
+ [
+ "2023-05-19T18:27:46",
+ 65149
+ ],
+ [
+ "2023-05-20T06:58:27",
+ 65247
+ ],
+ [
+ "2023-05-21T17:54:05",
+ 65353
+ ],
+ [
+ "2023-05-22T19:05:24",
+ 65540
+ ],
+ [
+ "2023-05-23T01:07:57",
+ 65598
+ ],
+ [
+ "2023-05-24T01:14:32",
+ 65843
+ ],
+ [
+ "2023-05-26T01:11:00",
+ 66471
+ ],
+ [
+ "2023-05-27T17:04:12",
+ 66727
+ ],
+ [
+ "2023-05-28T17:03:24",
+ 66788
+ ],
+ [
+ "2023-05-29T11:13:08",
+ 66872
+ ],
+ [
+ "2023-05-31T23:48:39",
+ 67518
+ ],
+ [
+ "2023-06-01T01:25:18",
+ 67540
+ ],
+ [
+ "2023-06-02T01:10:33",
+ 67746
+ ],
+ [
+ "2023-06-03T09:59:50",
+ 67948
+ ],
+ [
+ "2023-06-04T01:13:43",
+ 67991
+ ],
+ [
+ "2023-06-05T01:08:28",
+ 68043
+ ],
+ [
+ "2023-06-07T01:10:00",
+ 68684
+ ],
+ [
+ "2023-06-08T05:19:18",
+ 69031
+ ],
+ [
+ "2023-06-11T18:48:09",
+ 69579
+ ],
+ [
+ "2023-06-12T01:16:15",
+ 69631
+ ],
+ [
+ "2023-06-13T01:11:33",
+ 69939
+ ],
+ [
+ "2023-06-17T01:09:24",
+ 71103
+ ],
+ [
+ "2023-06-21T05:26:13",
+ 71933
+ ],
+ [
+ "2023-06-23T01:12:21",
+ 72425
+ ],
+ [
+ "2023-06-24T01:13:03",
+ 72723
+ ],
+ [
+ "2023-06-25T01:19:08",
+ 72767
+ ],
+ [
+ "2023-07-02T01:17:57",
+ 74840
+ ],
+ [
+ "2023-07-05T04:19:03",
+ 75591
+ ],
+ [
+ "2023-07-06T01:13:59",
+ 75909
+ ],
+ [
+ "2023-07-07T01:22:59",
+ 76220
+ ],
+ [
+ "2023-07-09T01:17:28",
+ 76549
+ ],
+ [
+ "2023-07-10T12:35:57",
+ 76815
+ ],
+ [
+ "2023-07-11T18:04:48",
+ 77401
+ ],
+ [
+ "2023-07-13T01:16:23",
+ 77844
+ ],
+ [
+ "2023-07-14T18:55:34",
+ 78446
+ ],
+ [
+ "2023-07-15T14:49:24",
+ 78520
+ ],
+ [
+ "2023-07-17T01:18:56",
+ 78617
]
],
"cupcake": [
@@ -3433,8 +3745,164 @@
10508
],
[
- "2023-05-14T16:41:46",
- 10516
+ "2023-05-14T19:54:23",
+ 10530
+ ],
+ [
+ "2023-05-17T23:06:02",
+ 10950
+ ],
+ [
+ "2023-05-18T18:05:13",
+ 11130
+ ],
+ [
+ "2023-05-19T18:27:46",
+ 11308
+ ],
+ [
+ "2023-05-20T06:58:27",
+ 11458
+ ],
+ [
+ "2023-05-21T17:54:05",
+ 11476
+ ],
+ [
+ "2023-05-22T19:05:24",
+ 11597
+ ],
+ [
+ "2023-05-23T01:07:57",
+ 11677
+ ],
+ [
+ "2023-05-24T01:14:32",
+ 11975
+ ],
+ [
+ "2023-05-26T01:11:00",
+ 12354
+ ],
+ [
+ "2023-05-27T17:04:12",
+ 12625
+ ],
+ [
+ "2023-05-28T17:03:24",
+ 12641
+ ],
+ [
+ "2023-05-29T11:13:08",
+ 12665
+ ],
+ [
+ "2023-05-31T23:48:39",
+ 13001
+ ],
+ [
+ "2023-06-01T01:25:18",
+ 13016
+ ],
+ [
+ "2023-06-02T01:10:33",
+ 13151
+ ],
+ [
+ "2023-06-03T09:59:50",
+ 13318
+ ],
+ [
+ "2023-06-04T01:13:43",
+ 13322
+ ],
+ [
+ "2023-06-05T01:08:28",
+ 13334
+ ],
+ [
+ "2023-06-07T01:10:00",
+ 13679
+ ],
+ [
+ "2023-06-08T05:19:18",
+ 13861
+ ],
+ [
+ "2023-06-11T18:48:09",
+ 14314
+ ],
+ [
+ "2023-06-12T01:16:15",
+ 14328
+ ],
+ [
+ "2023-06-13T01:11:33",
+ 14534
+ ],
+ [
+ "2023-06-17T01:09:24",
+ 15273
+ ],
+ [
+ "2023-06-21T05:26:13",
+ 15679
+ ],
+ [
+ "2023-06-23T01:12:21",
+ 16016
+ ],
+ [
+ "2023-06-24T01:13:03",
+ 16249
+ ],
+ [
+ "2023-06-25T01:19:08",
+ 16256
+ ],
+ [
+ "2023-07-02T01:17:57",
+ 17199
+ ],
+ [
+ "2023-07-05T04:19:03",
+ 17497
+ ],
+ [
+ "2023-07-06T01:13:59",
+ 17717
+ ],
+ [
+ "2023-07-07T01:22:59",
+ 17951
+ ],
+ [
+ "2023-07-09T01:17:28",
+ 18346
+ ],
+ [
+ "2023-07-10T12:35:57",
+ 18539
+ ],
+ [
+ "2023-07-11T18:04:48",
+ 18937
+ ],
+ [
+ "2023-07-13T01:16:23",
+ 19361
+ ],
+ [
+ "2023-07-14T18:55:34",
+ 19859
+ ],
+ [
+ "2023-07-15T14:49:24",
+ 19910
+ ],
+ [
+ "2023-07-17T01:18:56",
+ 20011
]
],
"dart": [
@@ -5829,42 +6297,198 @@
147866
],
[
- "2023-05-14T16:41:46",
- 147912
- ]
- ],
- "dotnet": [
+ "2023-05-14T19:54:23",
+ 147933
+ ],
[
- "2021-07-26T20:10:04",
- 215546
+ "2023-05-17T23:06:02",
+ 149017
],
[
- "2021-07-28T01:29:28",
- 215612
+ "2023-05-18T18:05:13",
+ 149270
],
[
- "2021-07-29T01:25:08",
- 215655
+ "2023-05-19T18:27:46",
+ 149612
],
[
- "2021-07-30T02:45:47",
- 215693
+ "2023-05-20T06:58:27",
+ 149753
],
[
- "2021-07-31T02:55:52",
- 215736
+ "2023-05-21T17:54:05",
+ 149917
],
[
- "2021-08-01T01:34:33",
- 215742
+ "2023-05-22T19:05:24",
+ 150249
],
[
- "2021-08-02T01:25:51",
- 215755
+ "2023-05-23T01:07:57",
+ 150318
],
[
- "2021-08-03T01:34:04",
- 215956
+ "2023-05-24T01:14:32",
+ 150695
+ ],
+ [
+ "2023-05-26T01:11:00",
+ 151632
+ ],
+ [
+ "2023-05-27T17:04:12",
+ 152034
+ ],
+ [
+ "2023-05-28T17:03:24",
+ 152191
+ ],
+ [
+ "2023-05-29T11:13:08",
+ 152305
+ ],
+ [
+ "2023-05-31T23:48:39",
+ 153372
+ ],
+ [
+ "2023-06-01T01:25:18",
+ 153427
+ ],
+ [
+ "2023-06-02T01:10:33",
+ 153773
+ ],
+ [
+ "2023-06-03T09:59:50",
+ 154155
+ ],
+ [
+ "2023-06-04T01:13:43",
+ 154230
+ ],
+ [
+ "2023-06-05T01:08:28",
+ 154337
+ ],
+ [
+ "2023-06-07T01:10:00",
+ 155093
+ ],
+ [
+ "2023-06-08T05:19:18",
+ 155542
+ ],
+ [
+ "2023-06-11T18:48:09",
+ 156562
+ ],
+ [
+ "2023-06-12T01:16:15",
+ 156602
+ ],
+ [
+ "2023-06-13T01:11:33",
+ 157113
+ ],
+ [
+ "2023-06-17T01:09:24",
+ 158705
+ ],
+ [
+ "2023-06-21T05:26:13",
+ 159755
+ ],
+ [
+ "2023-06-23T01:12:21",
+ 160416
+ ],
+ [
+ "2023-06-24T01:13:03",
+ 160693
+ ],
+ [
+ "2023-06-25T01:19:08",
+ 160764
+ ],
+ [
+ "2023-07-02T01:17:57",
+ 162559
+ ],
+ [
+ "2023-07-05T04:19:03",
+ 163036
+ ],
+ [
+ "2023-07-06T01:13:59",
+ 163294
+ ],
+ [
+ "2023-07-07T01:22:59",
+ 163534
+ ],
+ [
+ "2023-07-09T01:17:28",
+ 163815
+ ],
+ [
+ "2023-07-10T12:35:57",
+ 163986
+ ],
+ [
+ "2023-07-11T18:04:48",
+ 164354
+ ],
+ [
+ "2023-07-13T01:16:23",
+ 164673
+ ],
+ [
+ "2023-07-14T18:55:34",
+ 165267
+ ],
+ [
+ "2023-07-15T14:49:24",
+ 165378
+ ],
+ [
+ "2023-07-17T01:18:56",
+ 165480
+ ]
+ ],
+ "dotnet": [
+ [
+ "2021-07-26T20:10:04",
+ 215546
+ ],
+ [
+ "2021-07-28T01:29:28",
+ 215612
+ ],
+ [
+ "2021-07-29T01:25:08",
+ 215655
+ ],
+ [
+ "2021-07-30T02:45:47",
+ 215693
+ ],
+ [
+ "2021-07-31T02:55:52",
+ 215736
+ ],
+ [
+ "2021-08-01T01:34:33",
+ 215742
+ ],
+ [
+ "2021-08-02T01:25:51",
+ 215755
+ ],
+ [
+ "2021-08-03T01:34:04",
+ 215956
],
[
"2021-08-04T01:25:42",
@@ -7315,8 +7939,190 @@
383804
],
[
- "2023-05-14T16:41:46",
- 383850
+ "2023-05-14T19:54:23",
+ 383879
+ ],
+ [
+ "2023-05-17T23:06:02",
+ 385783
+ ],
+ [
+ "2023-05-18T18:05:13",
+ 386087
+ ],
+ [
+ "2023-05-19T18:27:46",
+ 386599
+ ],
+ [
+ "2023-05-20T06:58:27",
+ 386745
+ ],
+ [
+ "2023-05-21T17:54:05",
+ 386877
+ ],
+ [
+ "2023-05-22T19:05:24",
+ 387226
+ ],
+ [
+ "2023-05-23T01:07:57",
+ 387323
+ ],
+ [
+ "2023-05-24T01:14:32",
+ 387794
+ ],
+ [
+ "2023-05-26T01:11:00",
+ 388513
+ ],
+ [
+ "2023-05-27T17:04:12",
+ 388936
+ ],
+ [
+ "2023-05-28T17:03:24",
+ 389016
+ ],
+ [
+ "2023-05-29T11:13:08",
+ 389112
+ ],
+ [
+ "2023-05-31T23:48:39",
+ 390243
+ ],
+ [
+ "2023-06-01T01:25:18",
+ 390312
+ ],
+ [
+ "2023-06-02T01:10:33",
+ 390955
+ ],
+ [
+ "2023-06-03T09:59:50",
+ 391306
+ ],
+ [
+ "2023-06-04T01:13:43",
+ 391379
+ ],
+ [
+ "2023-06-05T01:08:28",
+ 391465
+ ],
+ [
+ "2023-06-07T01:10:00",
+ 392283
+ ],
+ [
+ "2023-06-08T05:19:18",
+ 392693
+ ],
+ [
+ "2023-06-11T18:48:09",
+ 393568
+ ],
+ [
+ "2023-06-12T01:16:15",
+ 393583
+ ],
+ [
+ "2023-06-13T01:11:33",
+ 394135
+ ],
+ [
+ "2023-06-17T01:09:24",
+ 395762
+ ],
+ [
+ "2023-06-21T05:26:13",
+ 396717
+ ],
+ [
+ "2023-06-23T01:12:21",
+ 397473
+ ],
+ [
+ "2023-06-24T01:13:03",
+ 397734
+ ],
+ [
+ "2023-06-25T01:19:08",
+ 397771
+ ],
+ [
+ "2023-07-02T01:17:57",
+ 399355
+ ],
+ [
+ "2023-07-05T04:19:03",
+ 399928
+ ],
+ [
+ "2023-07-06T01:13:59",
+ 400218
+ ],
+ [
+ "2023-07-07T01:22:59",
+ 400748
+ ],
+ [
+ "2023-07-09T01:17:28",
+ 401628
+ ],
+ [
+ "2023-07-10T12:35:57",
+ 402077
+ ],
+ [
+ "2023-07-11T18:04:48",
+ 403260
+ ],
+ [
+ "2023-07-13T01:16:23",
+ 404095
+ ],
+ [
+ "2023-07-14T18:55:34",
+ 405270
+ ],
+ [
+ "2023-07-15T14:49:24",
+ 405543
+ ],
+ [
+ "2023-07-17T01:18:56",
+ 405706
+ ]
+ ],
+ "dotnetweb": [
+ [
+ "2023-07-10T12:35:57",
+ 4
+ ],
+ [
+ "2023-07-11T18:04:48",
+ 16
+ ],
+ [
+ "2023-07-13T01:16:23",
+ 22
+ ],
+ [
+ "2023-07-14T18:55:34",
+ 30
+ ],
+ [
+ "2023-07-15T14:49:24",
+ 36
+ ],
+ [
+ "2023-07-17T01:18:56",
+ 38
]
],
"go": [
@@ -8801,8 +9607,164 @@
20443
],
[
- "2023-05-14T16:41:46",
- 20453
+ "2023-05-14T19:54:23",
+ 20467
+ ],
+ [
+ "2023-05-17T23:06:02",
+ 20680
+ ],
+ [
+ "2023-05-18T18:05:13",
+ 20760
+ ],
+ [
+ "2023-05-19T18:27:46",
+ 20837
+ ],
+ [
+ "2023-05-20T06:58:27",
+ 20865
+ ],
+ [
+ "2023-05-21T17:54:05",
+ 20874
+ ],
+ [
+ "2023-05-22T19:05:24",
+ 20974
+ ],
+ [
+ "2023-05-23T01:07:57",
+ 20992
+ ],
+ [
+ "2023-05-24T01:14:32",
+ 21082
+ ],
+ [
+ "2023-05-26T01:11:00",
+ 21221
+ ],
+ [
+ "2023-05-27T17:04:12",
+ 21294
+ ],
+ [
+ "2023-05-28T17:03:24",
+ 21334
+ ],
+ [
+ "2023-05-29T11:13:08",
+ 21381
+ ],
+ [
+ "2023-05-31T23:48:39",
+ 21592
+ ],
+ [
+ "2023-06-01T01:25:18",
+ 21604
+ ],
+ [
+ "2023-06-02T01:10:33",
+ 21672
+ ],
+ [
+ "2023-06-03T09:59:50",
+ 21713
+ ],
+ [
+ "2023-06-04T01:13:43",
+ 21717
+ ],
+ [
+ "2023-06-05T01:08:28",
+ 21731
+ ],
+ [
+ "2023-06-07T01:10:00",
+ 21899
+ ],
+ [
+ "2023-06-08T05:19:18",
+ 21991
+ ],
+ [
+ "2023-06-11T18:48:09",
+ 22116
+ ],
+ [
+ "2023-06-12T01:16:15",
+ 22124
+ ],
+ [
+ "2023-06-13T01:11:33",
+ 22228
+ ],
+ [
+ "2023-06-17T01:09:24",
+ 22516
+ ],
+ [
+ "2023-06-21T05:26:13",
+ 22719
+ ],
+ [
+ "2023-06-23T01:12:21",
+ 22866
+ ],
+ [
+ "2023-06-24T01:13:03",
+ 22940
+ ],
+ [
+ "2023-06-25T01:19:08",
+ 22946
+ ],
+ [
+ "2023-07-02T01:17:57",
+ 23373
+ ],
+ [
+ "2023-07-05T04:19:03",
+ 23570
+ ],
+ [
+ "2023-07-06T01:13:59",
+ 23644
+ ],
+ [
+ "2023-07-07T01:22:59",
+ 23756
+ ],
+ [
+ "2023-07-09T01:17:28",
+ 23898
+ ],
+ [
+ "2023-07-10T12:35:57",
+ 23975
+ ],
+ [
+ "2023-07-11T18:04:48",
+ 24107
+ ],
+ [
+ "2023-07-13T01:16:23",
+ 24222
+ ],
+ [
+ "2023-07-14T18:55:34",
+ 24382
+ ],
+ [
+ "2023-07-15T14:49:24",
+ 24395
+ ],
+ [
+ "2023-07-17T01:18:56",
+ 24424
]
],
"java": [
@@ -10251,44 +11213,200 @@
116906
],
[
- "2023-04-24T10:35:05",
- 116984
+ "2023-04-24T10:35:05",
+ 116984
+ ],
+ [
+ "2023-04-25T13:22:38",
+ 117126
+ ],
+ [
+ "2023-04-26T01:05:21",
+ 117202
+ ],
+ [
+ "2023-04-29T09:13:18",
+ 117528
+ ],
+ [
+ "2023-04-30T23:37:21",
+ 117596
+ ],
+ [
+ "2023-05-01T12:30:40",
+ 117623
+ ],
+ [
+ "2023-05-02T01:03:19",
+ 117682
+ ],
+ [
+ "2023-05-12T22:08:47",
+ 118821
+ ],
+ [
+ "2023-05-13T20:23:06",
+ 118907
+ ],
+ [
+ "2023-05-14T19:54:23",
+ 118965
+ ],
+ [
+ "2023-05-17T23:06:02",
+ 119365
+ ],
+ [
+ "2023-05-18T18:05:13",
+ 119481
+ ],
+ [
+ "2023-05-19T18:27:46",
+ 119605
+ ],
+ [
+ "2023-05-20T06:58:27",
+ 119649
+ ],
+ [
+ "2023-05-21T17:54:05",
+ 119746
+ ],
+ [
+ "2023-05-22T19:05:24",
+ 119861
+ ],
+ [
+ "2023-05-23T01:07:57",
+ 119886
+ ],
+ [
+ "2023-05-24T01:14:32",
+ 120045
+ ],
+ [
+ "2023-05-26T01:11:00",
+ 120330
+ ],
+ [
+ "2023-05-27T17:04:12",
+ 120494
+ ],
+ [
+ "2023-05-28T17:03:24",
+ 120553
+ ],
+ [
+ "2023-05-29T11:13:08",
+ 120588
+ ],
+ [
+ "2023-05-31T23:48:39",
+ 120936
+ ],
+ [
+ "2023-06-01T01:25:18",
+ 120955
+ ],
+ [
+ "2023-06-02T01:10:33",
+ 121071
+ ],
+ [
+ "2023-06-03T09:59:50",
+ 121214
+ ],
+ [
+ "2023-06-04T01:13:43",
+ 121249
+ ],
+ [
+ "2023-06-05T01:08:28",
+ 121309
+ ],
+ [
+ "2023-06-07T01:10:00",
+ 121707
+ ],
+ [
+ "2023-06-08T05:19:18",
+ 121904
+ ],
+ [
+ "2023-06-11T18:48:09",
+ 122298
+ ],
+ [
+ "2023-06-12T01:16:15",
+ 122329
+ ],
+ [
+ "2023-06-13T01:11:33",
+ 122526
+ ],
+ [
+ "2023-06-17T01:09:24",
+ 123348
+ ],
+ [
+ "2023-06-21T05:26:13",
+ 123866
+ ],
+ [
+ "2023-06-23T01:12:21",
+ 124267
+ ],
+ [
+ "2023-06-24T01:13:03",
+ 124501
+ ],
+ [
+ "2023-06-25T01:19:08",
+ 124559
+ ],
+ [
+ "2023-07-02T01:17:57",
+ 125975
+ ],
+ [
+ "2023-07-05T04:19:03",
+ 126405
],
[
- "2023-04-25T13:22:38",
- 117126
+ "2023-07-06T01:13:59",
+ 126628
],
[
- "2023-04-26T01:05:21",
- 117202
+ "2023-07-07T01:22:59",
+ 126827
],
[
- "2023-04-29T09:13:18",
- 117528
+ "2023-07-09T01:17:28",
+ 127130
],
[
- "2023-04-30T23:37:21",
- 117596
+ "2023-07-10T12:35:57",
+ 127358
],
[
- "2023-05-01T12:30:40",
- 117623
+ "2023-07-11T18:04:48",
+ 127681
],
[
- "2023-05-02T01:03:19",
- 117682
+ "2023-07-13T01:16:23",
+ 127977
],
[
- "2023-05-12T22:08:47",
- 118821
+ "2023-07-14T18:55:34",
+ 128391
],
[
- "2023-05-13T20:23:06",
- 118907
+ "2023-07-15T14:49:24",
+ 128461
],
[
- "2023-05-14T16:41:46",
- 118947
+ "2023-07-17T01:18:56",
+ 128530
]
],
"javascript": [
@@ -11773,8 +12891,164 @@
260231
],
[
- "2023-05-14T16:41:46",
- 260540
+ "2023-05-14T19:54:23",
+ 260734
+ ],
+ [
+ "2023-05-17T23:06:02",
+ 262757
+ ],
+ [
+ "2023-05-18T18:05:13",
+ 263290
+ ],
+ [
+ "2023-05-19T18:27:46",
+ 263955
+ ],
+ [
+ "2023-05-20T06:58:27",
+ 264160
+ ],
+ [
+ "2023-05-21T17:54:05",
+ 264649
+ ],
+ [
+ "2023-05-22T19:05:24",
+ 265504
+ ],
+ [
+ "2023-05-23T01:07:57",
+ 265671
+ ],
+ [
+ "2023-05-24T01:14:32",
+ 266335
+ ],
+ [
+ "2023-05-26T01:11:00",
+ 267622
+ ],
+ [
+ "2023-05-27T17:04:12",
+ 268377
+ ],
+ [
+ "2023-05-28T17:03:24",
+ 268641
+ ],
+ [
+ "2023-05-29T11:13:08",
+ 268976
+ ],
+ [
+ "2023-05-31T23:48:39",
+ 270723
+ ],
+ [
+ "2023-06-01T01:25:18",
+ 270848
+ ],
+ [
+ "2023-06-02T01:10:33",
+ 271657
+ ],
+ [
+ "2023-06-03T09:59:50",
+ 272295
+ ],
+ [
+ "2023-06-04T01:13:43",
+ 272391
+ ],
+ [
+ "2023-06-05T01:08:28",
+ 272653
+ ],
+ [
+ "2023-06-07T01:10:00",
+ 273929
+ ],
+ [
+ "2023-06-08T05:19:18",
+ 274617
+ ],
+ [
+ "2023-06-11T18:48:09",
+ 276437
+ ],
+ [
+ "2023-06-12T01:16:15",
+ 276584
+ ],
+ [
+ "2023-06-13T01:11:33",
+ 277578
+ ],
+ [
+ "2023-06-17T01:09:24",
+ 280668
+ ],
+ [
+ "2023-06-21T05:26:13",
+ 284231
+ ],
+ [
+ "2023-06-23T01:12:21",
+ 285611
+ ],
+ [
+ "2023-06-24T01:13:03",
+ 286468
+ ],
+ [
+ "2023-06-25T01:19:08",
+ 286651
+ ],
+ [
+ "2023-07-02T01:17:57",
+ 292703
+ ],
+ [
+ "2023-07-05T04:19:03",
+ 296096
+ ],
+ [
+ "2023-07-06T01:13:59",
+ 297315
+ ],
+ [
+ "2023-07-07T01:22:59",
+ 298646
+ ],
+ [
+ "2023-07-09T01:17:28",
+ 299698
+ ],
+ [
+ "2023-07-10T12:35:57",
+ 301182
+ ],
+ [
+ "2023-07-11T18:04:48",
+ 303260
+ ],
+ [
+ "2023-07-13T01:16:23",
+ 304595
+ ],
+ [
+ "2023-07-14T18:55:34",
+ 306496
+ ],
+ [
+ "2023-07-15T14:49:24",
+ 306845
+ ],
+ [
+ "2023-07-17T01:18:56",
+ 307377
]
],
"php": [
@@ -13259,8 +14533,164 @@
49374
],
[
- "2023-05-14T16:41:46",
- 49381
+ "2023-05-14T19:54:23",
+ 49393
+ ],
+ [
+ "2023-05-17T23:06:02",
+ 49529
+ ],
+ [
+ "2023-05-18T18:05:13",
+ 49564
+ ],
+ [
+ "2023-05-19T18:27:46",
+ 49635
+ ],
+ [
+ "2023-05-20T06:58:27",
+ 49654
+ ],
+ [
+ "2023-05-21T17:54:05",
+ 49663
+ ],
+ [
+ "2023-05-22T19:05:24",
+ 49704
+ ],
+ [
+ "2023-05-23T01:07:57",
+ 49708
+ ],
+ [
+ "2023-05-24T01:14:32",
+ 49750
+ ],
+ [
+ "2023-05-26T01:11:00",
+ 49869
+ ],
+ [
+ "2023-05-27T17:04:12",
+ 49926
+ ],
+ [
+ "2023-05-28T17:03:24",
+ 49930
+ ],
+ [
+ "2023-05-29T11:13:08",
+ 49939
+ ],
+ [
+ "2023-05-31T23:48:39",
+ 50079
+ ],
+ [
+ "2023-06-01T01:25:18",
+ 50083
+ ],
+ [
+ "2023-06-02T01:10:33",
+ 50168
+ ],
+ [
+ "2023-06-03T09:59:50",
+ 50256
+ ],
+ [
+ "2023-06-04T01:13:43",
+ 50274
+ ],
+ [
+ "2023-06-05T01:08:28",
+ 50290
+ ],
+ [
+ "2023-06-07T01:10:00",
+ 50424
+ ],
+ [
+ "2023-06-08T05:19:18",
+ 50506
+ ],
+ [
+ "2023-06-11T18:48:09",
+ 50690
+ ],
+ [
+ "2023-06-12T01:16:15",
+ 50693
+ ],
+ [
+ "2023-06-13T01:11:33",
+ 50761
+ ],
+ [
+ "2023-06-17T01:09:24",
+ 51059
+ ],
+ [
+ "2023-06-21T05:26:13",
+ 51184
+ ],
+ [
+ "2023-06-23T01:12:21",
+ 51246
+ ],
+ [
+ "2023-06-24T01:13:03",
+ 51289
+ ],
+ [
+ "2023-06-25T01:19:08",
+ 51291
+ ],
+ [
+ "2023-07-02T01:17:57",
+ 51514
+ ],
+ [
+ "2023-07-05T04:19:03",
+ 51573
+ ],
+ [
+ "2023-07-06T01:13:59",
+ 51619
+ ],
+ [
+ "2023-07-07T01:22:59",
+ 51638
+ ],
+ [
+ "2023-07-09T01:17:28",
+ 51687
+ ],
+ [
+ "2023-07-10T12:35:57",
+ 51714
+ ],
+ [
+ "2023-07-11T18:04:48",
+ 51775
+ ],
+ [
+ "2023-07-13T01:16:23",
+ 51854
+ ],
+ [
+ "2023-07-14T18:55:34",
+ 51951
+ ],
+ [
+ "2023-07-15T14:49:24",
+ 51963
+ ],
+ [
+ "2023-07-17T01:18:56",
+ 51965
]
],
"python": [
@@ -14741,12 +16171,168 @@
203644
],
[
- "2023-05-13T20:23:06",
- 203948
+ "2023-05-13T20:23:06",
+ 203948
+ ],
+ [
+ "2023-05-14T19:54:23",
+ 204309
+ ],
+ [
+ "2023-05-17T23:06:02",
+ 206008
+ ],
+ [
+ "2023-05-18T18:05:13",
+ 206554
+ ],
+ [
+ "2023-05-19T18:27:46",
+ 207221
+ ],
+ [
+ "2023-05-20T06:58:27",
+ 207495
+ ],
+ [
+ "2023-05-21T17:54:05",
+ 207701
+ ],
+ [
+ "2023-05-22T19:05:24",
+ 208159
+ ],
+ [
+ "2023-05-23T01:07:57",
+ 208311
+ ],
+ [
+ "2023-05-24T01:14:32",
+ 209103
+ ],
+ [
+ "2023-05-26T01:11:00",
+ 210357
+ ],
+ [
+ "2023-05-27T17:04:12",
+ 210946
+ ],
+ [
+ "2023-05-28T17:03:24",
+ 211066
+ ],
+ [
+ "2023-05-29T11:13:08",
+ 211275
+ ],
+ [
+ "2023-05-31T23:48:39",
+ 212640
+ ],
+ [
+ "2023-06-01T01:25:18",
+ 212752
+ ],
+ [
+ "2023-06-02T01:10:33",
+ 213377
+ ],
+ [
+ "2023-06-03T09:59:50",
+ 213971
+ ],
+ [
+ "2023-06-04T01:13:43",
+ 214027
+ ],
+ [
+ "2023-06-05T01:08:28",
+ 214127
+ ],
+ [
+ "2023-06-07T01:10:00",
+ 215444
+ ],
+ [
+ "2023-06-08T05:19:18",
+ 216241
+ ],
+ [
+ "2023-06-11T18:48:09",
+ 217500
+ ],
+ [
+ "2023-06-12T01:16:15",
+ 217578
+ ],
+ [
+ "2023-06-13T01:11:33",
+ 218129
+ ],
+ [
+ "2023-06-17T01:09:24",
+ 220479
+ ],
+ [
+ "2023-06-21T05:26:13",
+ 221931
+ ],
+ [
+ "2023-06-23T01:12:21",
+ 223162
+ ],
+ [
+ "2023-06-24T01:13:03",
+ 223567
+ ],
+ [
+ "2023-06-25T01:19:08",
+ 223616
+ ],
+ [
+ "2023-07-02T01:17:57",
+ 226462
+ ],
+ [
+ "2023-07-05T04:19:03",
+ 227461
+ ],
+ [
+ "2023-07-06T01:13:59",
+ 228077
+ ],
+ [
+ "2023-07-07T01:22:59",
+ 228529
+ ],
+ [
+ "2023-07-09T01:17:28",
+ 229209
+ ],
+ [
+ "2023-07-10T12:35:57",
+ 229620
+ ],
+ [
+ "2023-07-11T18:04:48",
+ 230515
+ ],
+ [
+ "2023-07-13T01:16:23",
+ 231337
+ ],
+ [
+ "2023-07-14T18:55:34",
+ 232317
+ ],
+ [
+ "2023-07-15T14:49:24",
+ 232549
],
[
- "2023-05-14T16:41:46",
- 204160
+ "2023-07-17T01:18:56",
+ 232726
]
],
"ruby": [
@@ -16227,8 +17813,164 @@
3557
],
[
- "2023-05-14T16:41:46",
- 3565
+ "2023-05-14T19:54:23",
+ 3577
+ ],
+ [
+ "2023-05-17T23:06:02",
+ 3583
+ ],
+ [
+ "2023-05-18T18:05:13",
+ 3589
+ ],
+ [
+ "2023-05-19T18:27:46",
+ 3601
+ ],
+ [
+ "2023-05-20T06:58:27",
+ 3603
+ ],
+ [
+ "2023-05-21T17:54:05",
+ 3603
+ ],
+ [
+ "2023-05-22T19:05:24",
+ 3606
+ ],
+ [
+ "2023-05-23T01:07:57",
+ 3606
+ ],
+ [
+ "2023-05-24T01:14:32",
+ 3613
+ ],
+ [
+ "2023-05-26T01:11:00",
+ 3621
+ ],
+ [
+ "2023-05-27T17:04:12",
+ 3640
+ ],
+ [
+ "2023-05-28T17:03:24",
+ 3644
+ ],
+ [
+ "2023-05-29T11:13:08",
+ 3647
+ ],
+ [
+ "2023-05-31T23:48:39",
+ 3660
+ ],
+ [
+ "2023-06-01T01:25:18",
+ 3663
+ ],
+ [
+ "2023-06-02T01:10:33",
+ 3666
+ ],
+ [
+ "2023-06-03T09:59:50",
+ 3672
+ ],
+ [
+ "2023-06-04T01:13:43",
+ 3674
+ ],
+ [
+ "2023-06-05T01:08:28",
+ 3676
+ ],
+ [
+ "2023-06-07T01:10:00",
+ 3680
+ ],
+ [
+ "2023-06-08T05:19:18",
+ 3684
+ ],
+ [
+ "2023-06-11T18:48:09",
+ 3690
+ ],
+ [
+ "2023-06-12T01:16:15",
+ 3693
+ ],
+ [
+ "2023-06-13T01:11:33",
+ 3697
+ ],
+ [
+ "2023-06-17T01:09:24",
+ 3733
+ ],
+ [
+ "2023-06-21T05:26:13",
+ 3766
+ ],
+ [
+ "2023-06-23T01:12:21",
+ 3768
+ ],
+ [
+ "2023-06-24T01:13:03",
+ 3770
+ ],
+ [
+ "2023-06-25T01:19:08",
+ 3772
+ ],
+ [
+ "2023-07-02T01:17:57",
+ 3779
+ ],
+ [
+ "2023-07-05T04:19:03",
+ 3789
+ ],
+ [
+ "2023-07-06T01:13:59",
+ 3792
+ ],
+ [
+ "2023-07-07T01:22:59",
+ 3804
+ ],
+ [
+ "2023-07-09T01:17:28",
+ 3808
+ ],
+ [
+ "2023-07-10T12:35:57",
+ 3816
+ ],
+ [
+ "2023-07-11T18:04:48",
+ 3828
+ ],
+ [
+ "2023-07-13T01:16:23",
+ 3844
+ ],
+ [
+ "2023-07-14T18:55:34",
+ 3860
+ ],
+ [
+ "2023-07-15T14:49:24",
+ 3866
+ ],
+ [
+ "2023-07-17T01:18:56",
+ 3868
]
],
"rust": [
@@ -17709,8 +19451,164 @@
5578
],
[
- "2023-05-14T16:41:46",
- 5586
+ "2023-05-14T19:54:23",
+ 5596
+ ],
+ [
+ "2023-05-17T23:06:02",
+ 5634
+ ],
+ [
+ "2023-05-18T18:05:13",
+ 5641
+ ],
+ [
+ "2023-05-19T18:27:46",
+ 5654
+ ],
+ [
+ "2023-05-20T06:58:27",
+ 5657
+ ],
+ [
+ "2023-05-21T17:54:05",
+ 5657
+ ],
+ [
+ "2023-05-22T19:05:24",
+ 5658
+ ],
+ [
+ "2023-05-23T01:07:57",
+ 5658
+ ],
+ [
+ "2023-05-24T01:14:32",
+ 5658
+ ],
+ [
+ "2023-05-26T01:11:00",
+ 5674
+ ],
+ [
+ "2023-05-27T17:04:12",
+ 5699
+ ],
+ [
+ "2023-05-28T17:03:24",
+ 5703
+ ],
+ [
+ "2023-05-29T11:13:08",
+ 5705
+ ],
+ [
+ "2023-05-31T23:48:39",
+ 5743
+ ],
+ [
+ "2023-06-01T01:25:18",
+ 5746
+ ],
+ [
+ "2023-06-02T01:10:33",
+ 5776
+ ],
+ [
+ "2023-06-03T09:59:50",
+ 5790
+ ],
+ [
+ "2023-06-04T01:13:43",
+ 5792
+ ],
+ [
+ "2023-06-05T01:08:28",
+ 5795
+ ],
+ [
+ "2023-06-07T01:10:00",
+ 5806
+ ],
+ [
+ "2023-06-08T05:19:18",
+ 5818
+ ],
+ [
+ "2023-06-11T18:48:09",
+ 5839
+ ],
+ [
+ "2023-06-12T01:16:15",
+ 5842
+ ],
+ [
+ "2023-06-13T01:11:33",
+ 5858
+ ],
+ [
+ "2023-06-17T01:09:24",
+ 5889
+ ],
+ [
+ "2023-06-21T05:26:13",
+ 5967
+ ],
+ [
+ "2023-06-23T01:12:21",
+ 6017
+ ],
+ [
+ "2023-06-24T01:13:03",
+ 6035
+ ],
+ [
+ "2023-06-25T01:19:08",
+ 6037
+ ],
+ [
+ "2023-07-02T01:17:57",
+ 6096
+ ],
+ [
+ "2023-07-05T04:19:03",
+ 6143
+ ],
+ [
+ "2023-07-06T01:13:59",
+ 6153
+ ],
+ [
+ "2023-07-07T01:22:59",
+ 6164
+ ],
+ [
+ "2023-07-09T01:17:28",
+ 6176
+ ],
+ [
+ "2023-07-10T12:35:57",
+ 6187
+ ],
+ [
+ "2023-07-11T18:04:48",
+ 6206
+ ],
+ [
+ "2023-07-13T01:16:23",
+ 6233
+ ],
+ [
+ "2023-07-14T18:55:34",
+ 6255
+ ],
+ [
+ "2023-07-15T14:49:24",
+ 6262
+ ],
+ [
+ "2023-07-17T01:18:56",
+ 6266
]
],
"salesforce": [
@@ -19183,20 +21081,176 @@
18859
],
[
- "2023-05-02T01:03:19",
- 18876
+ "2023-05-02T01:03:19",
+ 18876
+ ],
+ [
+ "2023-05-12T22:08:47",
+ 19292
+ ],
+ [
+ "2023-05-13T20:23:06",
+ 19321
+ ],
+ [
+ "2023-05-14T19:54:23",
+ 19349
+ ],
+ [
+ "2023-05-17T23:06:02",
+ 19561
+ ],
+ [
+ "2023-05-18T18:05:13",
+ 19677
+ ],
+ [
+ "2023-05-19T18:27:46",
+ 19748
+ ],
+ [
+ "2023-05-20T06:58:27",
+ 19761
+ ],
+ [
+ "2023-05-21T17:54:05",
+ 19780
+ ],
+ [
+ "2023-05-22T19:05:24",
+ 19806
+ ],
+ [
+ "2023-05-23T01:07:57",
+ 19813
+ ],
+ [
+ "2023-05-24T01:14:32",
+ 19874
+ ],
+ [
+ "2023-05-26T01:11:00",
+ 19987
+ ],
+ [
+ "2023-05-27T17:04:12",
+ 20060
+ ],
+ [
+ "2023-05-28T17:03:24",
+ 20076
+ ],
+ [
+ "2023-05-29T11:13:08",
+ 20089
+ ],
+ [
+ "2023-05-31T23:48:39",
+ 20240
+ ],
+ [
+ "2023-06-01T01:25:18",
+ 20249
+ ],
+ [
+ "2023-06-02T01:10:33",
+ 20299
+ ],
+ [
+ "2023-06-03T09:59:50",
+ 20348
+ ],
+ [
+ "2023-06-04T01:13:43",
+ 20352
+ ],
+ [
+ "2023-06-05T01:08:28",
+ 20361
+ ],
+ [
+ "2023-06-07T01:10:00",
+ 20421
+ ],
+ [
+ "2023-06-08T05:19:18",
+ 20454
+ ],
+ [
+ "2023-06-11T18:48:09",
+ 20563
+ ],
+ [
+ "2023-06-12T01:16:15",
+ 20573
+ ],
+ [
+ "2023-06-13T01:11:33",
+ 20610
+ ],
+ [
+ "2023-06-17T01:09:24",
+ 20763
+ ],
+ [
+ "2023-06-21T05:26:13",
+ 20859
+ ],
+ [
+ "2023-06-23T01:12:21",
+ 20923
+ ],
+ [
+ "2023-06-24T01:13:03",
+ 20947
+ ],
+ [
+ "2023-06-25T01:19:08",
+ 20960
+ ],
+ [
+ "2023-07-02T01:17:57",
+ 21229
+ ],
+ [
+ "2023-07-05T04:19:03",
+ 21351
+ ],
+ [
+ "2023-07-06T01:13:59",
+ 21394
+ ],
+ [
+ "2023-07-07T01:22:59",
+ 21446
+ ],
+ [
+ "2023-07-09T01:17:28",
+ 21481
+ ],
+ [
+ "2023-07-10T12:35:57",
+ 21522
+ ],
+ [
+ "2023-07-11T18:04:48",
+ 21589
+ ],
+ [
+ "2023-07-13T01:16:23",
+ 21642
],
[
- "2023-05-12T22:08:47",
- 19292
+ "2023-07-14T18:55:34",
+ 21712
],
[
- "2023-05-13T20:23:06",
- 19321
+ "2023-07-15T14:49:24",
+ 21727
],
[
- "2023-05-14T16:41:46",
- 19339
+ "2023-07-17T01:18:56",
+ 21741
]
],
"scala": [
@@ -20679,8 +22733,164 @@
9125
],
[
- "2023-05-14T16:41:46",
- 9131
+ "2023-05-14T19:54:23",
+ 9139
+ ],
+ [
+ "2023-05-17T23:06:02",
+ 9367
+ ],
+ [
+ "2023-05-18T18:05:13",
+ 9391
+ ],
+ [
+ "2023-05-19T18:27:46",
+ 9423
+ ],
+ [
+ "2023-05-20T06:58:27",
+ 9425
+ ],
+ [
+ "2023-05-21T17:54:05",
+ 9425
+ ],
+ [
+ "2023-05-22T19:05:24",
+ 9629
+ ],
+ [
+ "2023-05-23T01:07:57",
+ 9666
+ ],
+ [
+ "2023-05-24T01:14:32",
+ 9920
+ ],
+ [
+ "2023-05-26T01:11:00",
+ 10646
+ ],
+ [
+ "2023-05-27T17:04:12",
+ 10996
+ ],
+ [
+ "2023-05-28T17:03:24",
+ 11028
+ ],
+ [
+ "2023-05-29T11:13:08",
+ 11127
+ ],
+ [
+ "2023-05-31T23:48:39",
+ 11861
+ ],
+ [
+ "2023-06-01T01:25:18",
+ 11923
+ ],
+ [
+ "2023-06-02T01:10:33",
+ 12363
+ ],
+ [
+ "2023-06-03T09:59:50",
+ 12567
+ ],
+ [
+ "2023-06-04T01:13:43",
+ 12589
+ ],
+ [
+ "2023-06-05T01:08:28",
+ 12635
+ ],
+ [
+ "2023-06-07T01:10:00",
+ 13067
+ ],
+ [
+ "2023-06-08T05:19:18",
+ 13248
+ ],
+ [
+ "2023-06-11T18:48:09",
+ 13693
+ ],
+ [
+ "2023-06-12T01:16:15",
+ 13734
+ ],
+ [
+ "2023-06-13T01:11:33",
+ 14118
+ ],
+ [
+ "2023-06-17T01:09:24",
+ 14849
+ ],
+ [
+ "2023-06-21T05:26:13",
+ 15511
+ ],
+ [
+ "2023-06-23T01:12:21",
+ 16060
+ ],
+ [
+ "2023-06-24T01:13:03",
+ 16321
+ ],
+ [
+ "2023-06-25T01:19:08",
+ 16342
+ ],
+ [
+ "2023-07-02T01:17:57",
+ 17810
+ ],
+ [
+ "2023-07-05T04:19:03",
+ 18325
+ ],
+ [
+ "2023-07-06T01:13:59",
+ 18553
+ ],
+ [
+ "2023-07-07T01:22:59",
+ 18849
+ ],
+ [
+ "2023-07-09T01:17:28",
+ 19004
+ ],
+ [
+ "2023-07-10T12:35:57",
+ 19221
+ ],
+ [
+ "2023-07-11T18:04:48",
+ 19473
+ ],
+ [
+ "2023-07-13T01:16:23",
+ 19652
+ ],
+ [
+ "2023-07-14T18:55:34",
+ 20018
+ ],
+ [
+ "2023-07-15T14:49:24",
+ 20096
+ ],
+ [
+ "2023-07-17T01:18:56",
+ 20204
]
],
"swift": [
@@ -22161,8 +24371,164 @@
3698
],
[
- "2023-05-14T16:41:46",
- 3704
+ "2023-05-14T19:54:23",
+ 3710
+ ],
+ [
+ "2023-05-17T23:06:02",
+ 3724
+ ],
+ [
+ "2023-05-18T18:05:13",
+ 3734
+ ],
+ [
+ "2023-05-19T18:27:46",
+ 3747
+ ],
+ [
+ "2023-05-20T06:58:27",
+ 3750
+ ],
+ [
+ "2023-05-21T17:54:05",
+ 3750
+ ],
+ [
+ "2023-05-22T19:05:24",
+ 3752
+ ],
+ [
+ "2023-05-23T01:07:57",
+ 3754
+ ],
+ [
+ "2023-05-24T01:14:32",
+ 3770
+ ],
+ [
+ "2023-05-26T01:11:00",
+ 3778
+ ],
+ [
+ "2023-05-27T17:04:12",
+ 3797
+ ],
+ [
+ "2023-05-28T17:03:24",
+ 3801
+ ],
+ [
+ "2023-05-29T11:13:08",
+ 3804
+ ],
+ [
+ "2023-05-31T23:48:39",
+ 3831
+ ],
+ [
+ "2023-06-01T01:25:18",
+ 3834
+ ],
+ [
+ "2023-06-02T01:10:33",
+ 3841
+ ],
+ [
+ "2023-06-03T09:59:50",
+ 3851
+ ],
+ [
+ "2023-06-04T01:13:43",
+ 3853
+ ],
+ [
+ "2023-06-05T01:08:28",
+ 3855
+ ],
+ [
+ "2023-06-07T01:10:00",
+ 3867
+ ],
+ [
+ "2023-06-08T05:19:18",
+ 3879
+ ],
+ [
+ "2023-06-11T18:48:09",
+ 3900
+ ],
+ [
+ "2023-06-12T01:16:15",
+ 3903
+ ],
+ [
+ "2023-06-13T01:11:33",
+ 3911
+ ],
+ [
+ "2023-06-17T01:09:24",
+ 3962
+ ],
+ [
+ "2023-06-21T05:26:13",
+ 3980
+ ],
+ [
+ "2023-06-23T01:12:21",
+ 4003
+ ],
+ [
+ "2023-06-24T01:13:03",
+ 4006
+ ],
+ [
+ "2023-06-25T01:19:08",
+ 4008
+ ],
+ [
+ "2023-07-02T01:17:57",
+ 4066
+ ],
+ [
+ "2023-07-05T04:19:03",
+ 4077
+ ],
+ [
+ "2023-07-06T01:13:59",
+ 4086
+ ],
+ [
+ "2023-07-07T01:22:59",
+ 4089
+ ],
+ [
+ "2023-07-09T01:17:28",
+ 4094
+ ],
+ [
+ "2023-07-10T12:35:57",
+ 4104
+ ],
+ [
+ "2023-07-11T18:04:48",
+ 4111
+ ],
+ [
+ "2023-07-13T01:16:23",
+ 4128
+ ],
+ [
+ "2023-07-14T18:55:34",
+ 4149
+ ],
+ [
+ "2023-07-15T14:49:24",
+ 4153
+ ],
+ [
+ "2023-07-17T01:18:56",
+ 4155
]
],
"terraform": [
@@ -23647,8 +26013,164 @@
235593
],
[
- "2023-05-14T16:41:46",
- 235771
+ "2023-05-14T19:54:23",
+ 235814
+ ],
+ [
+ "2023-05-17T23:06:02",
+ 238459
+ ],
+ [
+ "2023-05-18T18:05:13",
+ 239156
+ ],
+ [
+ "2023-05-19T18:27:46",
+ 239948
+ ],
+ [
+ "2023-05-20T06:58:27",
+ 240232
+ ],
+ [
+ "2023-05-21T17:54:05",
+ 240531
+ ],
+ [
+ "2023-05-22T19:05:24",
+ 241277
+ ],
+ [
+ "2023-05-23T01:07:57",
+ 241474
+ ],
+ [
+ "2023-05-24T01:14:32",
+ 242491
+ ],
+ [
+ "2023-05-26T01:11:00",
+ 244365
+ ],
+ [
+ "2023-05-27T17:04:12",
+ 245380
+ ],
+ [
+ "2023-05-28T17:03:24",
+ 245717
+ ],
+ [
+ "2023-05-29T11:13:08",
+ 245977
+ ],
+ [
+ "2023-05-31T23:48:39",
+ 248209
+ ],
+ [
+ "2023-06-01T01:25:18",
+ 248323
+ ],
+ [
+ "2023-06-02T01:10:33",
+ 249165
+ ],
+ [
+ "2023-06-03T09:59:50",
+ 249997
+ ],
+ [
+ "2023-06-04T01:13:43",
+ 250124
+ ],
+ [
+ "2023-06-05T01:08:28",
+ 250347
+ ],
+ [
+ "2023-06-07T01:10:00",
+ 252116
+ ],
+ [
+ "2023-06-08T05:19:18",
+ 253143
+ ],
+ [
+ "2023-06-11T18:48:09",
+ 254925
+ ],
+ [
+ "2023-06-12T01:16:15",
+ 255039
+ ],
+ [
+ "2023-06-13T01:11:33",
+ 255828
+ ],
+ [
+ "2023-06-17T01:09:24",
+ 259200
+ ],
+ [
+ "2023-06-21T05:26:13",
+ 261514
+ ],
+ [
+ "2023-06-23T01:12:21",
+ 262906
+ ],
+ [
+ "2023-06-24T01:13:03",
+ 263681
+ ],
+ [
+ "2023-06-25T01:19:08",
+ 263966
+ ],
+ [
+ "2023-07-02T01:17:57",
+ 269312
+ ],
+ [
+ "2023-07-05T04:19:03",
+ 271231
+ ],
+ [
+ "2023-07-06T01:13:59",
+ 271949
+ ],
+ [
+ "2023-07-07T01:22:59",
+ 272589
+ ],
+ [
+ "2023-07-09T01:17:28",
+ 273517
+ ],
+ [
+ "2023-07-10T12:35:57",
+ 274119
+ ],
+ [
+ "2023-07-11T18:04:48",
+ 275604
+ ],
+ [
+ "2023-07-13T01:16:23",
+ 276730
+ ],
+ [
+ "2023-07-14T18:55:34",
+ 278381
+ ],
+ [
+ "2023-07-15T14:49:24",
+ 278757
+ ],
+ [
+ "2023-07-17T01:18:56",
+ 279222
]
]
}
\ No newline at end of file
diff --git a/.automation/generated/linter-helps.json b/.automation/generated/linter-helps.json
index a187dc895f9..9f22902efbb 100644
--- a/.automation/generated/linter-helps.json
+++ b/.automation/generated/linter-helps.json
@@ -130,7 +130,7 @@
" -w WARN_LIST, --warn-list WARN_LIST",
" only warn about these rules, unless overridden in",
" config file. Current version default value is:",
- " experimental, jinja[spacing]",
+ " experimental, jinja[spacing], fqcn[deep]",
" --enable-list ENABLE_LIST",
" activate optional rules by their tag name",
" --nocolor disable colored output, same as NO_COLOR=1",
@@ -140,7 +140,8 @@
" repeatable.",
" -c CONFIG_FILE, --config-file CONFIG_FILE",
" Specify configuration file to use. By default it will",
- " look for '.ansible-lint' or '.config/ansible-lint.yml'",
+ " look for '.ansible-lint', '.config/ansible-lint.yml',",
+ " or '.config/ansible-lint.yaml'",
" -i IGNORE_FILE, --ignore-file IGNORE_FILE",
" Specify ignore file to use. By default it will look",
" for '.ansible-lint-ignore' or '.config/ansible-lint-",
@@ -369,7 +370,7 @@
"General help using GNU software: "
],
"bicep_linter": [
- "Bicep CLI version 0.17.1 (d423d61882)",
+ "Bicep CLI version 0.19.5 (87ca110fc0)",
"",
"Usage:",
" bicep build [options] ",
@@ -379,10 +380,11 @@
" The input file",
"",
" Options:",
- " --outdir Saves the output at the specified directory.",
- " --outfile Saves the output as the specified file path.",
- " --stdout Prints the output to stdout.",
- " --no-restore Builds the bicep file without restoring external modules.",
+ " --outdir Saves the output at the specified directory.",
+ " --outfile Saves the output as the specified file path.",
+ " --stdout Prints the output to stdout.",
+ " --no-restore Builds the bicep file without restoring external modules.",
+ " --diagnostics-format Sets the format with which diagnostics are displayed. Valid values are ( Default | Sarif ).",
"",
" Examples:",
" bicep build file.bicep",
@@ -390,6 +392,7 @@
" bicep build file.bicep --outdir dir1",
" bicep build file.bicep --outfile file.json",
" bicep build file.bicep --no-restore",
+ " bicep build file.bicep --diagnostics-format sarif",
"",
" bicep format [options] ",
" Formats a .bicep file.",
@@ -423,7 +426,7 @@
" --outdir Saves the output at the specified directory.",
" --outfile Saves the output as the specified file path.",
" --stdout Prints the output to stdout.",
- " --force Allows overwriting the output file if it exists (applies only to 'bicep decompile').",
+ " --force Allows overwriting the output file if it exists (applies only to 'bicep decompile' or 'bicep decompile-params').",
"",
" Examples:",
" bicep decompile file.json",
@@ -432,8 +435,29 @@
" bicep decompile file.json --force",
" bicep decompile file.json --outfile file.bicep",
"",
+ " bicep decompile-params [options] ",
+ " Attempts to decompile a parameters .json file to .bicepparam.",
+ "",
+ " Arguments:",
+ " The input file",
+ "",
+ " Options:",
+ " --outdir Saves the output at the specified directory.",
+ " --outfile Saves the output as the specified file path.",
+ " --stdout Prints the output to stdout.",
+ " --force Allows overwriting the output file if it exists (applies only to 'bicep decompile' or 'bicep decompile-params').",
+ " --bicep-file Path to the bicep template file (relative to the .bicepparam file) that will be referenced in the using declaration",
+ "",
+ " Examples:",
+ " bicep decompile-params file.json",
+ " bicep decompile-params file.json --bicep-file ./dir/main.bicep",
+ " bicep decompile-params file.json --stdout",
+ " bicep decompile-params file.json --outdir dir1",
+ " bicep decompile-params file.json --force",
+ " bicep decompile-params file.json --outfile file.bicepparam",
+ "",
" bicep generate-params [options] ",
- " Builds .parameters.json file from the given bicep file, updates if there is an existing parameters.json file.",
+ " Builds parameters file from the given bicep file, updates if there is an existing parameters file.",
"",
" Arguments:",
" The input file",
@@ -443,6 +467,8 @@
" --outdir Saves the output at the specified directory.",
" --outfile Saves the output as the specified file path.",
" --stdout Prints the output to stdout.",
+ " --output-format Selects the output format {json, bicepparam}",
+ " --include-params Selects which parameters to include into output {requiredonly, all}",
"",
" Examples:",
" bicep generate-params file.bicep",
@@ -450,7 +476,7 @@
" bicep generate-params file.bicep --stdout",
" bicep generate-params file.bicep --outdir dir1",
" bicep generate-params file.bicep --outfile file.parameters.json",
- "",
+ " bicep generate-params file.bicep --output-format bicepparam --include-params all",
"",
" bicep publish --target [",
" Publishes the .bicep file to the module registry.",
@@ -475,16 +501,15 @@
" Arguments:",
" The input file",
"",
- " bicep [options]",
+ " bicep [options]",
" Options:",
" --version -v Shows bicep version information",
" --help -h Shows this usage information",
" --license Prints license information",
" --third-party-notices Prints third-party notices",
"",
- "",
" bicep build-params ",
- " Builds .bicepparam file.",
+ " Builds a .json file from a .bicepparam file.",
"",
" Arguments:",
" The input Bicepparam file",
@@ -500,7 +525,6 @@
" bicep build-params params.bicepparam --stdout",
" bicep build-params params.bicepparam --outfile otherParams.json",
" bicep build-params params.bicepparam --no-restore",
- "",
""
],
"black": [
@@ -512,7 +536,7 @@
" -c, --code TEXT Format the code passed in as a string.",
" -l, --line-length INTEGER How many characters per line to allow.",
" [default: 88]",
- " -t, --target-version [py33|py34|py35|py36|py37|py38|py39|py310|py311]",
+ " -t, --target-version [py33|py34|py35|py36|py37|py38|py39|py310|py311|py312]",
" Python versions that should be supported by",
" Black's output. By default, Black will try",
" to infer this from the project metadata in",
@@ -571,9 +595,10 @@
" directories on all platforms (Windows, too).",
" Exclusions are calculated first, inclusions",
" later. [default: /(\\.direnv|\\.eggs|\\.git|\\.h",
- " g|\\.mypy_cache|\\.nox|\\.tox|\\.venv|venv|\\.svn",
- " |\\.ipynb_checkpoints|_build|buck-",
- " out|build|dist|__pypackages__)/]",
+ " g|\\.ipynb_checkpoints|\\.mypy_cache|\\.nox|\\.p",
+ " ytest_cache|\\.ruff_cache|\\.tox|\\.svn|\\.venv|",
+ " \\.vscode|__pypackages__|_build|buck-",
+ " out|build|dist|venv)/]",
" --extend-exclude TEXT Like --exclude, but adds additional files",
" and directories on top of the excluded ones.",
" (Useful if you simply want to add to the",
@@ -586,8 +611,9 @@
" stdin. Useful to make sure Black will",
" respect --force-exclude option on some",
" editors that rely on using stdin.",
- " -W, --workers INTEGER RANGE Number of parallel workers [default: number",
- " of CPUs in the system] [x>=1]",
+ " -W, --workers INTEGER RANGE Number of parallel workers [default:",
+ " BLACK_NUM_WORKERS environment variable or",
+ " number of CPUs in the system] [x>=1]",
" -q, --quiet Don't emit non-error messages to stderr.",
" Errors are still emitted; silence those with",
" 2>/dev/null.",
@@ -602,7 +628,7 @@
"usage:",
"Basic: cfn-lint test.yaml",
"Ignore a rule: cfn-lint -i E3012 -- test.yaml",
- "Configure a rule: cfn-lint -x E3012:strict=false -t test.yaml",
+ "Configure a rule: cfn-lint -x E3012:strict=true -t test.yaml",
"Lint all yaml files in a folder: cfn-lint dir/**/*.yaml",
"",
"CloudFormation Linter",
@@ -634,7 +660,7 @@
" Include experimental rules",
" -x CONFIGURE_RULES [CONFIGURE_RULES ...], --configure-rule CONFIGURE_RULES [CONFIGURE_RULES ...]",
" Provide configuration for a rule. Format",
- " RuleId:key=value. Example: E3012:strict=false",
+ " RuleId:key=value. Example: E3012:strict=true",
" --config-file CONFIG_FILE",
" Specify the cfnlintrc file to use",
" -z CUSTOM_RULES, --custom-rules CUSTOM_RULES",
@@ -682,11 +708,11 @@
" --list-rules List registered rules"
],
"checkov": [
- "usage: checkov [-h] [-v] [--support] [-d DIRECTORY] [--add-check] [-f FILE]",
- " [--skip-path SKIP_PATH]",
+ "usage: checkov [-h] [-v] [--support] [-d DIRECTORY] [--add-check]",
+ " [-f FILE [FILE ...]] [--skip-path SKIP_PATH]",
" [--external-checks-dir EXTERNAL_CHECKS_DIR]",
" [--external-checks-git EXTERNAL_CHECKS_GIT] [-l]",
- " [-o {cli,cyclonedx,cyclonedx_json,json,junitxml,github_failed_only,gitlab_sast,sarif,csv}]",
+ " [-o {cli,csv,cyclonedx,cyclonedx_json,json,junitxml,github_failed_only,gitlab_sast,sarif,spdx}]",
" [--output-file-path OUTPUT_FILE_PATH] [--output-bc-ids]",
" [--include-all-checkov-policies] [--quiet] [--compact]",
" [--framework {ansible,argo_workflows,arm,azure_pipelines,bicep,bitbucket_pipelines,circleci_pipelines,cloudformation,dockerfile,github_configuration,github_actions,gitlab_configuration,gitlab_ci,bitbucket_configuration,helm,json,yaml,kubernetes,kustomize,openapi,sca_package,sca_image,secrets,serverless,terraform,terraform_json,terraform_plan,3d_policy,all} [{ansible,argo_workflows,arm,azure_pipelines,bicep,bitbucket_pipelines,circleci_pipelines,cloudformation,dockerfile,github_configuration,github_actions,gitlab_configuration,gitlab_ci,bitbucket_configuration,helm,json,yaml,kubernetes,kustomize,openapi,sca_package,sca_image,secrets,serverless,terraform,terraform_json,terraform_plan,3d_policy,all} ...]]",
@@ -694,7 +720,8 @@
" [-c CHECK] [--skip-check SKIP_CHECK]",
" [--run-all-external-checks] [-s] [--soft-fail-on SOFT_FAIL_ON]",
" [--hard-fail-on HARD_FAIL_ON] [--bc-api-key BC_API_KEY]",
- " [--prisma-api-url PRISMA_API_URL] [--docker-image DOCKER_IMAGE]",
+ " [--prisma-api-url PRISMA_API_URL] [--skip-results-upload]",
+ " [--docker-image DOCKER_IMAGE]",
" [--dockerfile-path DOCKERFILE_PATH] [--repo-id REPO_ID]",
" [-b BRANCH] [--skip-download] [--use-enforcement-rules]",
" [--no-guide] [--skip-suppressions] [--skip-policy-download]",
@@ -730,7 +757,8 @@
" IaC root directory (can not be used together with",
" --file).",
" --add-check Generate a new check via CLI prompt",
- " -f FILE, --file FILE File to scan (can not be used together with",
+ " -f FILE [FILE ...], --file FILE [FILE ...]",
+ " File to scan (can not be used together with",
" --directory). With this option, Checkov will attempt",
" to filter the runners based on the file type. For",
" example, if you specify a \".tf\" file, only the",
@@ -752,7 +780,7 @@
" specify a subdirectory after a double-slash //. cannot",
" be used together with --external-checks-dir",
" -l, --list List checks",
- " -o {cli,cyclonedx,cyclonedx_json,json,junitxml,github_failed_only,gitlab_sast,sarif,csv}, --output {cli,cyclonedx,cyclonedx_json,json,junitxml,github_failed_only,gitlab_sast,sarif,csv}",
+ " -o {cli,csv,cyclonedx,cyclonedx_json,json,junitxml,github_failed_only,gitlab_sast,sarif,spdx}, --output {cli,csv,cyclonedx,cyclonedx_json,json,junitxml,github_failed_only,gitlab_sast,sarif,spdx}",
" Report output format. Add multiple outputs by using",
" the flag multiple times (-o sarif -o cli)",
" --output-file-path OUTPUT_FILE_PATH",
@@ -872,6 +900,11 @@
" --bc-api-key to be a Prisma Cloud Access Key in the",
" following format: :: [env",
" var: PRISMA_API_URL]",
+ " --skip-results-upload",
+ " Do not upload scan results to the platform to view in",
+ " the console. Results are only available locally. If",
+ " you use the --support flag, logs will still get",
+ " uploaded.",
" --docker-image DOCKER_IMAGE, --image DOCKER_IMAGE",
" Scan docker images by name or ID. Only works with",
" --bc-api-key flag",
@@ -1007,13 +1040,12 @@
" receive enhanced guidelines using",
" CKV_OPENAI_MAX_FINDINGS [env var: CKV_OPENAI_API_KEY]",
"",
- "Args that start with '--' (eg. -v) can also be set in a config file",
- "(/.checkov.yaml or /.checkov.yml or /root/.checkov.yaml or /root/.checkov.yml",
- "or specified via --config-file). The config file uses YAML syntax and must",
- "represent a YAML 'mapping' (for details, see",
- "http://learn.getgrav.org/advanced/yaml). If an arg is specified in more than",
- "one place, then commandline values override environment variables which",
- "override config file values which override defaults."
+ "Args that start with '--' can also be set in a config file (/.checkov.yaml or",
+ "/.checkov.yml or /root/.checkov.yaml or /root/.checkov.yml or specified via",
+ "--config-file). The config file uses YAML syntax and must represent a YAML",
+ "'mapping' (for details, see http://learn.getgrav.org/advanced/yaml). In",
+ "general, command-line values override environment variables which override",
+ "config file values which override defaults."
],
"checkstyle": [
"Usage: java [options] [args...]",
@@ -1193,12 +1225,12 @@
"",
"Common options:",
" --no-deps Run Clippy only on the given crate, without linting the dependencies",
- " --fix Automatically apply lint suggestions. This flag implies `--no-deps`",
+ " --fix Automatically apply lint suggestions. This flag implies `--no-deps` and `--all-targets`",
" -h, --help Print this message",
" -V, --version Print version info and exit",
" --explain LINT Print the documentation for a given lint",
"",
- "Other options are the same as `cargo check`.",
+ "For the other options see `cargo check --help`.",
"",
"To allow or deny a lint from the command line you can use `cargo clippy --`",
"with:",
@@ -1214,7 +1246,7 @@
""
],
"clj-kondo": [
- "clj-kondo v2023.04.14",
+ "clj-kondo v2023.07.13",
"",
"",
"Options:",
@@ -1237,7 +1269,7 @@
" nearest `.clj-kondo` directory in the current and parent directories.",
"",
" --config : config may be a file or an EDN expression. See",
- " https://cljdoc.org/d/clj-kondo/clj-kondo/2023.04.14/doc/configuration",
+ " https://cljdoc.org/d/clj-kondo/clj-kondo/2023.07.13/doc/configuration",
"",
" --config-dir : use this config directory instead of auto-detected",
" .clj-kondo dir.",
@@ -1528,7 +1560,9 @@
"",
"Options:",
" --check Check that files are formatted. Will not write any changes.",
+ " --loglevel Specify the log level - Debug, Information (default), Warning, Error, None [default: Information]",
" --no-cache Bypass the cache to determine if a file needs to be formatted.",
+ " --no-msbuild-check Bypass the check to determine if a csproj files references a different version of CSharpier.MsBuild.",
" --fast Skip comparing syntax tree of formatted file to original file to validate changes.",
" --skip-write Skip writing changes. Generally used for testing to ensure csharpier doesn't throw any errors or cause syntax tree validation failures.",
" --write-stdout Write the results of formatting any files to stdout.",
@@ -1587,22 +1621,20 @@
""
],
"devskim": [
- "Microsoft DevSkim Command Line Interface 0.7.104+bd34d6c82b",
+ "devskim 1.0.11+87ad45b866",
+ "\u00a9 Microsoft Corporation. All rights reserved.",
"",
- "Usage: devskim [options] [command]",
+ " analyze Analyze source code using DevSkim",
"",
- "Options:",
- " -?|-h|--help Show help information",
- " -v|--version Show version information",
+ " fix Apply fixes from a Sarif",
"",
- "Commands:",
- " analyze Analyze source code",
- " catalogue Create csv file catalogue of rules",
- " pack Pack rules into a single file",
- " test Run tests for rules",
- " verify Verify integrity and syntax of rules",
+ " verify Verify rule validity",
+ "",
+ " suppress Suppress issues identified in a DevSkim Sarif",
"",
- "Use \"devskim [command] --help\" for more information about a command.",
+ " help Display more information on a specific command.",
+ "",
+ " version Display version information.",
""
],
"djlint": [
@@ -1661,6 +1693,12 @@
" --indent-js INTEGER Set JS indent level.",
" --close-void-tags Add closing mark on known void tags. Ex:",
" ]
becomse
",
+ " --no-line-after-yaml Do not add a blank line after yaml front",
+ " matter.",
+ " --no-function-formatting Do not attempt to format function contents.",
+ " --no-set-formatting Do not attempt to format set contents.",
+ " --max-blank-lines INTEGER Consolidate blank lines down to x lines.",
+ " [default: 0]",
" -h, --help Show this message and exit."
],
"dockerfilelint": [
@@ -2118,7 +2156,7 @@
" --no-banner suppress banner",
" --no-color turn off color for verbose output",
" --redact redact secrets from logs and stdout",
- " -f, --report-format string output format (json, csv, sarif) (default \"json\")",
+ " -f, --report-format string output format (json, csv, junit, sarif) (default \"json\")",
" -r, --report-path string report file",
" -s, --source string path to source (default \".\")",
" -v, --verbose show verbose output from scan",
@@ -2182,6 +2220,60 @@
" --version output the version number",
" -h, --help output usage information"
],
+ "grype": [
+ "A vulnerability scanner for container images, filesystems, and SBOMs.",
+ "",
+ "Supports the following image sources:",
+ " grype yourrepo/yourimage:tag defaults to using images from a Docker daemon",
+ " grype path/to/yourproject a Docker tar, OCI tar, OCI directory, SIF container, or generic filesystem directory",
+ "",
+ "You can also explicitly specify the scheme to use:",
+ " grype podman:yourrepo/yourimage:tag explicitly use the Podman daemon",
+ " grype docker:yourrepo/yourimage:tag explicitly use the Docker daemon",
+ " grype docker-archive:path/to/yourimage.tar use a tarball from disk for archives created from \"docker save\"",
+ " grype oci-archive:path/to/yourimage.tar use a tarball from disk for OCI archives (from Podman or otherwise)",
+ " grype oci-dir:path/to/yourimage read directly from a path on disk for OCI layout directories (from Skopeo or otherwise)",
+ " grype singularity:path/to/yourimage.sif read directly from a Singularity Image Format (SIF) container on disk",
+ " grype dir:path/to/yourproject read directly from a path on disk (any directory)",
+ " grype sbom:path/to/syft.json read Syft JSON from path on disk",
+ " grype registry:yourrepo/yourimage:tag pull image directly from a registry (no container runtime required)",
+ " grype purl:path/to/purl/file read a newline separated file of purls from a path on disk",
+ "",
+ "You can also pipe in Syft JSON directly:",
+ " syft yourimage:tag -o json | grype",
+ "",
+ "Usage:",
+ " grype [IMAGE] [flags]",
+ " grype [command]",
+ "",
+ "Available Commands:",
+ " completion Generate a shell completion for Grype (listing local docker images)",
+ " db vulnerability database operations",
+ " help Help about any command",
+ " version show the version",
+ "",
+ "Flags:",
+ " --add-cpes-if-none generate CPEs for packages with no CPE data",
+ " --by-cve orient results by CVE instead of the original vulnerability ID when possible",
+ " -c, --config string application config file",
+ " --distro string distro to match against in the format: :",
+ " --exclude stringArray exclude paths from being scanned using a glob expression",
+ " -f, --fail-on string set the return code to 1 if a vulnerability is found with a severity >= the given severity, options=[negligible low medium high critical]",
+ " --file string file to write the report output to (default is STDOUT)",
+ " -h, --help help for grype",
+ " --name string set the name of the target being analyzed",
+ " --only-fixed ignore matches for vulnerabilities that are not fixed",
+ " --only-notfixed ignore matches for vulnerabilities that are fixed",
+ " -o, --output string report output formatter, formats=[json table cyclonedx cyclonedx-json sarif template], deprecated formats=[embedded-cyclonedx-vex-json embedded-cyclonedx-vex-xml]",
+ " --platform string an optional platform specifier for container image sources (e.g. 'linux/arm64', 'linux/arm64/v8', 'arm64', 'linux')",
+ " -q, --quiet suppress all logging output",
+ " -s, --scope string selection of layers to analyze, options=[Squashed AllLayers] (default \"Squashed\")",
+ " --show-suppressed show suppressed/ignored vulnerabilities in the output (only supported with table output format)",
+ " -t, --template string specify the path to a Go template file (requires 'template' output to be selected)",
+ " -v, --verbose count increase verbosity (-v = info, -vv = debug)",
+ "",
+ "Use \"grype [command] --help\" for more information about a command."
+ ],
"hadolint": [
"hadolint - Dockerfile Linter written in Haskell",
"",
@@ -2923,7 +3015,7 @@
" Comma-separated list of rules to globally disable. To",
" disable standard ktlint rule-set use",
" --disabled_rules=standard",
- " -F, --format Fix any deviations from the code style",
+ " -F, --format Fix deviations from the code style when possible",
" --limit= Maximum number of errors to show (default: show all)",
" --relative Print files relative to the working directory (e.g.",
" dir/file.kt instead of /home/user/project/dir/file.kt)",
@@ -2985,7 +3077,7 @@
" -n int",
" number of goroutines to run concurrently (default 4)",
" -output string",
- " output format - json, junit, tap, text (default \"text\")",
+ " output format - json, junit, pretty, tap, text (default \"text\")",
" -reject string",
" comma-separated list of kinds or GVKs to reject",
" -schema-location value",
@@ -3001,6 +3093,49 @@
" print results for all resources (ignored for tap and junit output)",
""
],
+ "kubescape": [
+ "Kubescape is a tool for testing Kubernetes security posture. Docs: https://hub.armosec.io/docs",
+ "",
+ "Usage:",
+ " kubescape [command]",
+ "",
+ "Examples:",
+ "",
+ " # Scan command",
+ " kubescape scan",
+ "",
+ " # List supported frameworks",
+ " kubescape list frameworks",
+ "",
+ " # Download artifacts (air-gapped environment support)",
+ " kubescape download artifacts",
+ "",
+ " # View cached configurations",
+ " kubescape config view",
+ "",
+ "",
+ "Available Commands:",
+ " completion Generate autocompletion script",
+ " config Handle cached configurations",
+ " delete Delete configurations in Kubescape SaaS version",
+ " download Download exceptions,control,framework,artifacts,attack-tracks,controls-inputs",
+ " fix Fix misconfiguration in files",
+ " help Help about any command",
+ " list List frameworks/controls will list the supported frameworks and controls",
+ " scan Scan the current running cluster or yaml files",
+ " submit Submit an object to the Kubescape SaaS version",
+ " update Update your version",
+ " version Get current version",
+ "",
+ "Flags:",
+ " --cache-dir string Cache directory [$KS_CACHE_DIR] (default \"/root/.kubescape\")",
+ " --disable-color Disable Color output for logging",
+ " --enable-color Force enable Color output for logging",
+ " -h, --help help for kubescape",
+ " -l, --logger string Logger level. Supported: debug/info/success/warning/error/fatal [$KS_LOGGER] (default \"info\")",
+ "",
+ "Use \"kubescape [command] --help\" for more information about a command."
+ ],
"kubeval": [
"Validate a Kubernetes YAML file against the relevant schema",
"",
@@ -3056,7 +3191,7 @@
" [--exclude-files [] ...]",
" [--include-files [] ...]",
"",
- "luacheck 1.1.0, a linter and a static analyzer for Lua.",
+ "luacheck 1.1.1, a linter and a static analyzer for Lua.",
"",
"Arguments:",
" files List of files, directories and rockspecs to check. Pass",
@@ -3220,6 +3355,173 @@
" Luacheck on GitHub: https://github.com/lunarmodules/luacheck",
" Luacheck documentation: https://luacheck.readthedocs.org"
],
+ "lychee": [
+ "A fast, async link checker",
+ "",
+ "Finds broken URLs and mail addresses inside Markdown, HTML, `reStructuredText`, websites and more!",
+ "",
+ "Usage: lychee [OPTIONS] ...",
+ "",
+ "Arguments:",
+ " ...",
+ " The inputs (where to get links to check from). These can be: files (e.g. `README.md`), file globs (e.g. `\"~/git/*/README.md\"`), remote URLs (e.g. `https://example.com/README.md`) or standard input (`-`). NOTE: Use `--` to separate inputs from options that allow multiple arguments",
+ "",
+ "Options:",
+ " -c, --config ",
+ " Configuration file to use",
+ "",
+ " [default: lychee.toml]",
+ "",
+ " -v, --verbose...",
+ " Set verbosity level; more output per occurrence (e.g. `-v` or `-vv`)",
+ "",
+ " -q, --quiet...",
+ " Less output per occurrence (e.g. `-q` or `-qq`)",
+ "",
+ " -n, --no-progress",
+ " Do not show progress bar.",
+ " This is recommended for non-interactive shells (e.g. for continuous integration)",
+ "",
+ " --cache",
+ " Use request cache stored on disk at `.lycheecache`",
+ "",
+ " --max-cache-age ",
+ " Discard all cached requests older than this duration",
+ "",
+ " [default: 1d]",
+ "",
+ " --dump",
+ " Don't perform any link checking. Instead, dump all the links extracted from inputs that would be checked",
+ "",
+ " --archive ",
+ " Specify the use of a specific web archive. Can be used in combination with `--suggest`",
+ "",
+ " [possible values: wayback]",
+ "",
+ " --suggest",
+ " Suggest link replacements for broken links, using a web archive. The web archive can be specified with `--archive`",
+ "",
+ " -m, --max-redirects ",
+ " Maximum number of allowed redirects",
+ "",
+ " [default: 5]",
+ "",
+ " --max-retries ",
+ " Maximum number of retries per request",
+ "",
+ " [default: 3]",
+ "",
+ " --max-concurrency ",
+ " Maximum number of concurrent network requests",
+ "",
+ " [default: 128]",
+ "",
+ " -T, --threads ",
+ " Number of threads to utilize. Defaults to number of cores available to the system",
+ "",
+ " -u, --user-agent ",
+ " User agent",
+ "",
+ " [default: lychee/0.13.0]",
+ "",
+ " -i, --insecure",
+ " Proceed for server connections considered insecure (invalid TLS)",
+ "",
+ " -s, --scheme ",
+ " Only test links with the given schemes (e.g. http and https)",
+ "",
+ " --offline",
+ " Only check local files and block network requests",
+ "",
+ " --include ",
+ " URLs to check (supports regex). Has preference over all excludes",
+ "",
+ " --exclude ",
+ " Exclude URLs and mail addresses from checking (supports regex)",
+ "",
+ " --exclude-file ",
+ " Deprecated; use `--exclude-path` instead",
+ "",
+ " --exclude-path ",
+ " Exclude file path from getting checked",
+ "",
+ " -E, --exclude-all-private",
+ " Exclude all private IPs from checking.",
+ " Equivalent to `--exclude-private --exclude-link-local --exclude-loopback`",
+ "",
+ " --exclude-private",
+ " Exclude private IP address ranges from checking",
+ "",
+ " --exclude-link-local",
+ " Exclude link-local IP address range from checking",
+ "",
+ " --exclude-loopback",
+ " Exclude loopback IP address range and localhost from checking",
+ "",
+ " --exclude-mail",
+ " Exclude all mail addresses from checking",
+ "",
+ " --remap ",
+ " Remap URI matching pattern to different URI",
+ "",
+ " --header ",
+ " Custom request header",
+ "",
+ " -a, --accept ",
+ " Comma-separated list of accepted status codes for valid links",
+ "",
+ " -t, --timeout ",
+ " Website timeout in seconds from connect to response finished",
+ "",
+ " [default: 20]",
+ "",
+ " -r, --retry-wait-time ",
+ " Minimum wait time in seconds between retries of failed requests",
+ "",
+ " [default: 1]",
+ "",
+ " -X, --method ",
+ " Request method",
+ "",
+ " [default: get]",
+ "",
+ " -b, --base ",
+ " Base URL or website root directory to check relative URLs e.g. https://example.com or `/path/to/public`",
+ "",
+ " --basic-auth ",
+ " Basic authentication support. E.g. `username:password`",
+ "",
+ " --github-token ",
+ " GitHub API token to use when checking github.com links, to avoid rate limiting",
+ "",
+ " [env: GITHUB_TOKEN]",
+ "",
+ " --skip-missing",
+ " Skip missing input files (default is to error if they don't exist)",
+ "",
+ " --include-verbatim",
+ " Find links in verbatim sections like `pre`- and `code` blocks",
+ "",
+ " --glob-ignore-case",
+ " Ignore case when expanding filesystem path glob inputs",
+ "",
+ " -o, --output